prompt
stringlengths 2
14.7k
| completion
stringlengths 1
99.7k
|
---|---|
--wl.MaxVelocity = 0.007
--wl.Part0 = script.Parent.Parent.Misc.FL.Door.WD
--wl.Part1 = wl.Parent | |
--[[Weight and CG]] |
Tune.Weight = 1100 -- Total weight (in pounds)
Tune.WeightBSize = { -- Size of weight brick (dimmensions in studs ; larger = more stable)
--[[Width]] 6.5 ,
--[[Height]] 4.8 ,
--[[Length]] 17 }
Tune.WeightDist = 51 -- Weight distribution (0 - on rear wheels, 100 - on front wheels, can be <0 or >100)
Tune.CGHeight = .7 -- Center of gravity height (studs relative to median of all wheels)
Tune.WBVisible = false -- Makes the weight brick visible
|
-- Existance in this list signifies that it is an emote, the value indicates if it is a looping emote |
local emoteNames = { wave = false, point = false, dance = true, dance2 = true, dance3 = true, laugh = false, cheer = false}
math.randomseed(tick())
function configureAnimationSet(name, fileList)
if animTable[name] then
for _, connection in pairs(animTable[name].connections) do
connection:disconnect()
end
end
animTable[name] = {}
animTable[name].count = 0
animTable[name].totalWeight = 0
animTable[name].connections = {}
-- check for config values
local config = script:FindFirstChild(name)
if config then |
--//Server Animations |
RightHighReady = CFrame.new(-1, 0.55, -1.1) * CFrame.Angles(math.rad(-90), math.rad(0), math.rad(0));
LeftHighReady = CFrame.new(1,0.55,-1.15) * CFrame.Angles(math.rad(-90),math.rad(0),math.rad(0));
RightLowReady = CFrame.new(-1, 0.55, -1.1) * CFrame.Angles(math.rad(-90), math.rad(0), math.rad(0));
LeftLowReady = CFrame.new(1,0.55,-1.15) * CFrame.Angles(math.rad(-90),math.rad(0),math.rad(0));
RightPatrol = CFrame.new(-1, 0.55, -1.1) * CFrame.Angles(math.rad(-90), math.rad(0), math.rad(0));
LeftPatrol = CFrame.new(1,0.55,-1.15) * CFrame.Angles(math.rad(-90),math.rad(0),math.rad(0));
RightAim = CFrame.new(-1, 0.55, -1.1) * CFrame.Angles(math.rad(-90), math.rad(0), math.rad(0));
LeftAim = CFrame.new(1,0.55,-1.15) * CFrame.Angles(math.rad(-90),math.rad(0),math.rad(0));
RightSprint = CFrame.new(-1, 0.55, -1.1) * CFrame.Angles(math.rad(-90), math.rad(0), math.rad(0));
LeftSprint = CFrame.new(1,0.55,-1.15) * CFrame.Angles(math.rad(-90),math.rad(0),math.rad(0));
ShootPos = CFrame.new(0,0,.25);
}
return module
|
-- Replacements for RootCamera:RotateCamera() which did not actually rotate the camera
-- suppliedLookVector is not normally passed in, it's used only by Watch camera |
function BaseCamera:CalculateNewLookCFrame(suppliedLookVector)
local currLookVector = suppliedLookVector or self:GetCameraLookVector()
local currPitchAngle = math.asin(currLookVector.y)
local yTheta = math.clamp(self.rotateInput.y, -MAX_Y + currPitchAngle, -MIN_Y + currPitchAngle)
local constrainedRotateInput = Vector2.new(self.rotateInput.x, yTheta)
local startCFrame = CFrame.new(ZERO_VECTOR3, currLookVector)
local newLookCFrame = CFrame.Angles(0, -constrainedRotateInput.x, 0) * startCFrame * CFrame.Angles(-constrainedRotateInput.y,0,0)
return newLookCFrame
end
function BaseCamera:CalculateNewLookVector(suppliedLookVector)
local newLookCFrame = self:CalculateNewLookCFrame(suppliedLookVector)
return newLookCFrame.lookVector
end
function BaseCamera:CalculateNewLookVectorVR()
local subjectPosition = self:GetSubjectPosition()
local vecToSubject = (subjectPosition - game.Workspace.CurrentCamera.CFrame.p)
local currLookVector = (vecToSubject * X1_Y0_Z1).unit
local vrRotateInput = Vector2.new(self.rotateInput.x, 0)
local startCFrame = CFrame.new(ZERO_VECTOR3, currLookVector)
local yawRotatedVector = (CFrame.Angles(0, -vrRotateInput.x, 0) * startCFrame * CFrame.Angles(-vrRotateInput.y,0,0)).lookVector
return (yawRotatedVector * X1_Y0_Z1).unit
end
function BaseCamera:GetHumanoid()
local character = player and player.Character
if character then
local resultHumanoid = self.humanoidCache[player]
if resultHumanoid and resultHumanoid.Parent == character then
return resultHumanoid
else
self.humanoidCache[player] = nil -- Bust Old Cache
local humanoid = character:FindFirstChildOfClass("Humanoid")
if humanoid then
self.humanoidCache[player] = humanoid
end
return humanoid
end
end
return nil
end
function BaseCamera:GetHumanoidPartToFollow(humanoid, humanoidStateType)
if humanoidStateType == Enum.HumanoidStateType.Dead then
local character = humanoid.Parent
if character then
return character:FindFirstChild("Head") or humanoid.Torso
else
return humanoid.Torso
end
else
return humanoid.Torso
end
end
function BaseCamera:UpdateGamepad()
local gamepadPan = self.gamepadPanningCamera
if gamepadPan and (self.hasGameLoaded or not VRService.VREnabled) then
gamepadPan = Util.GamepadLinearToCurve(gamepadPan)
local currentTime = tick()
if gamepadPan.X ~= 0 or gamepadPan.Y ~= 0 then
self.userPanningTheCamera = true
elseif gamepadPan == ZERO_VECTOR2 then
if FFlagUserFixGamepadCameraTracking then
self.userPanningTheCamera = false
self.gamepadPanningCamera = false
end
self.lastThumbstickRotate = nil
if self.lastThumbstickPos == ZERO_VECTOR2 then
self.currentSpeed = 0
end
end
local finalConstant = 0
if self.lastThumbstickRotate then
if VRService.VREnabled then
self.currentSpeed = self.vrMaxSpeed
else
local elapsedTime = (currentTime - self.lastThumbstickRotate) * 10
self.currentSpeed = self.currentSpeed + (self.maxSpeed * ((elapsedTime*elapsedTime)/self.numOfSeconds))
if self.currentSpeed > self.maxSpeed then self.currentSpeed = self.maxSpeed end
if self.lastVelocity then
local velocity = (gamepadPan - self.lastThumbstickPos)/(currentTime - self.lastThumbstickRotate)
local velocityDeltaMag = (velocity - self.lastVelocity).magnitude
if velocityDeltaMag > 12 then
self.currentSpeed = self.currentSpeed * (20/velocityDeltaMag)
if self.currentSpeed > self.maxSpeed then self.currentSpeed = self.maxSpeed end
end
end
end
finalConstant = UserGameSettings.GamepadCameraSensitivity * self.currentSpeed
self.lastVelocity = (gamepadPan - self.lastThumbstickPos)/(currentTime - self.lastThumbstickRotate)
end
self.lastThumbstickPos = gamepadPan
self.lastThumbstickRotate = currentTime
return Vector2.new( gamepadPan.X * finalConstant, gamepadPan.Y * finalConstant * self.ySensitivity * UserGameSettings:GetCameraYInvertValue())
end
return ZERO_VECTOR2
end
|
--[[
Function called upon entering the state
]] |
function PlayerGame.onEnter(stateMachine, serverPlayer, event, from, to)
local player = serverPlayer.player
coroutine.wrap(
function()
local character = player.Character
if not character or not character.PrimaryPart then
player:LoadCharacter()
character = player.Character or player.CharacterAdded:Wait()
while not character.PrimaryPart do
wait()
end
character:WaitForChild("Humanoid")
end
if from ~= "camera" then
onCharacterAdded(player, character)
end
local humanoid = character:FindFirstChild("Humanoid")
humanoid.WalkSpeed = 16
local primaryPart = character.PrimaryPart
connections.characterAdded =
player.CharacterAdded:Connect(
function(character)
while not character.Parent or character:FindFirstChild("Humanoid") do
wait()
end
onCharacterAdded(player, character)
end
)
while stateMachine.current == "game" do
if isMoving then
local currentTick = tick()
MonsterManager.createInterestWhileTrue(
primaryPart.Position,
NOISE_GENERATED,
function()
return not isMoving or tick() < currentTick + NOISE_TIMEOUT
end
)
end
wait(0.25)
end
end
)()
end
|
-- Here be dragons
-- luacheck: ignore 212 |
local GuiService = game:GetService("GuiService")
local UserInputService = game:GetService("UserInputService")
local Players = game:GetService("Players")
local Player = Players.LocalPlayer
local LINE_HEIGHT = 20
local WINDOW_MAX_HEIGHT = 300
|
--[[Engine]] |
--Torque Curve
Tune.Horsepower = 560 -- [TORQUE CURVE VISUAL]
Tune.IdleRPM = 500 -- https://www.desmos.com/calculator/2uo3hqwdhf
Tune.PeakRPM = 3900 -- Use sliders to manipulate values
Tune.Redline = 4500 -- Copy and paste slider values into the respective tune values
Tune.EqPoint = 4500
Tune.PeakSharpness = 7.5
Tune.CurveMult = 0.16
--Incline Compensation
Tune.InclineComp = 1.3 -- Torque compensation multiplier for inclines (applies gradient from 0-90 degrees)
--Misc
Tune.RevAccel = 80 -- RPM acceleration when clutch is off
Tune.RevDecay = 70 -- RPM decay when clutch is off
Tune.RevBounce = 10 -- RPM kickback from redline
Tune.IdleThrottle = 1 -- Percent throttle at idle
Tune.ClutchTol = 500 -- Clutch engagement threshold (higher = faster response)
|
-- |
sp=script.Parent
sp.Luminate.SourceValueChanged:connect(function(val)
if val==1 then
sp.Transparency=0
sp.Sparkles.Enabled=true
elseif val==0 then
sp.Transparency=.5
sp.Sparkles.Enabled=false
end
end)
|
--[[[Default Controls]] |
--Peripheral Deadzones
Tune.Peripherals = {
MSteerWidth = 67 , -- Mouse steering control width (0 - 100% of screen width)
MSteerDZone = 10 , -- Mouse steering deadzone (0 - 100%)
ControlLDZone = 5 , -- Controller steering L-deadzone (0 - 100%)
ControlRDZone = 5 , -- Controller steering R-deadzone (0 - 100%)
}
--Control Mapping
Tune.Controls = {
--Keyboard Controls
--Mode Toggles
ToggleTCS = Enum.KeyCode.T ,
ToggleABS = Enum.KeyCode.Y ,
ToggleTransMode = Enum.KeyCode.M ,
--Primary Controls
Throttle = Enum.KeyCode.Up ,
Brake = Enum.KeyCode.Down ,
SteerLeft = Enum.KeyCode.Left ,
SteerRight = Enum.KeyCode.Right ,
--Secondary Controls
Throttle2 = Enum.KeyCode.W ,
Brake2 = Enum.KeyCode.S ,
SteerLeft2 = Enum.KeyCode.A ,
SteerRight2 = Enum.KeyCode.D ,
--Manual Transmission
ShiftUp = Enum.KeyCode.E ,
ShiftDown = Enum.KeyCode.Q ,
Clutch = Enum.KeyCode.LeftShift ,
--Handbrake
PBrake = Enum.KeyCode.P ,
--Mouse Controls
MouseThrottle = Enum.UserInputType.MouseButton1 ,
MouseBrake = Enum.UserInputType.MouseButton2 ,
MouseClutch = Enum.KeyCode.W ,
MouseShiftUp = Enum.KeyCode.E ,
MouseShiftDown = Enum.KeyCode.Q ,
MousePBrake = Enum.KeyCode.LeftShift ,
--Controller Mapping
ContlrThrottle = Enum.KeyCode.ButtonR2 ,
ContlrBrake = Enum.KeyCode.ButtonL2 ,
ContlrSteer = Enum.KeyCode.Thumbstick1 ,
ContlrShiftUp = Enum.KeyCode.ButtonY ,
ContlrShiftDown = Enum.KeyCode.ButtonX ,
ContlrClutch = Enum.KeyCode.ButtonR1 ,
ContlrPBrake = Enum.KeyCode.ButtonL1 ,
ContlrToggleTMode = Enum.KeyCode.DPadUp ,
ContlrToggleTCS = Enum.KeyCode.DPadDown ,
ContlrToggleABS = Enum.KeyCode.DPadRight ,
}
|
--AudioLib.__index = function(s, i) return s[i] end |
function AudioLib.new(container)
local self = {}
self.DefaultProperties = {
Children = nil;
--ClearAllChildren = true;
Looped = false;
PlaybackSpeed = 1;
Playing = false;
SoundId = nil;
TimePosition = 0;
Volume = 0.25;
}
self.Playlist = {}
self.Shuffle = false
function self:GetSound() -- Retrieve the sound used for playing all music
return self.Sound
end
function self:UpdateSound(data) -- Loops through a table of properties used to overwrite those of an existing instance.
for property,value in pairs(data) do
self.Sound[property] = value
end
return self.Sound
end
function self:SoundEnded() -- Loads the next song in the playlist
if #self.Playlist > 0 then
local index = table.find(self.Playlist, self.Sound.SoundId)
if self.Shuffle then index = math.random(0, #self.Playlist) end
if (index + 1) > #self.Playlist then
index = 0
end
self.Sound.SoundId = self.Playlist[index + 1]
end
end
local container = workspace:FindFirstChild(container.Name)
self.Sound = container:FindFirstChild("AudioLib_Sound")
if not self.Sound then
self.Sound = Instance.new("Sound")
self.Sound.Name = "AudioLib_Sound"
self.Sound.Parent = container -- Don't ask me why I have to do this.
self.Sound.Ended:Connect(function() self:SoundEnded() end)
self:UpdateSound(self.DefaultProperties)
end
return self
end
return AudioLib
|
--Defining the parts of the shelf |
local sp=script.Parent
local drawer=sp.DrawerModel.Drawer
local val = script.bool.Value
function onClicked(clicked)
if val == true then
val = false
drawer.ClickDetector.MaxActivationDistance=0
drawer.Sound:Play()
for loop = 1, 20 do
sp:SetPrimaryPartCFrame(sp.PrimaryPart.CFrame * CFrame.new(0, 0,-.1) * CFrame.fromEulerAnglesXYZ(0, 0, 0))
wait(0.05)
end
val = false
drawer.ClickDetector.MaxActivationDistance=5
elseif val == false then
val = true
drawer.ClickDetector.MaxActivationDistance=0
drawer.Sound:Play()
for loop = 1, 20 do
sp:SetPrimaryPartCFrame(sp.PrimaryPart.CFrame * CFrame.new(0, 0, .1) * CFrame.fromEulerAnglesXYZ(0, 0, 0))
wait(0.05)
end
val = true
drawer.ClickDetector.MaxActivationDistance=5
end
end
drawer.ClickDetector.MouseClick:connect(onClicked)
|
-- Basic Settings |
AdminCredit=false; -- Enables the credit GUI for that appears in the bottom right
AutoClean=false; -- Enables automatic cleaning of hats & tools in the Workspace
AutoCleanDelay=60; -- The delay between each AutoClean routine
CommandBar=true; -- Enables the Command Bar | GLOBAL KEYBIND: \
FunCommands=true; -- Enables fun yet unnecessary commands
FreeAdmin=false; -- Set to 1-5 to grant admin powers to all, otherwise set to false
PublicLogs=false; -- Allows all users to see the command & chat logs
Prefix=';'; -- Character to begin a command
--[[
Admin Powers
0 Player
1 VIP Can use nonabusive commands only on self
2 Moderator Can kick, mute, & use most commands
3 Administrator Can ban, crash, & set Moderators/VIP
4 SuperAdmin Can grant permanent powers, & shutdown the game
5 Owner Can set SuperAdmins, & use all the commands
6 Game Creator Can set owners & use all the commands
Group & VIP Admin
You can set multiple Groups & Ranks to grant users admin powers:
GroupAdmin={
[12345]={[254]=4,[253]=3};
[GROUPID]={[RANK]=ADMINPOWER}
};
You can set multiple Assets to grant users admin powers:
VIPAdmin={
[12345]=3;
[54321]=4;
[ITEMID]=ADMINPOWER;
}; ]]
GroupAdmin={
};
VIPAdmin={
};
|
--// initialize variables //-- |
local playerTable = nil
local viewingNumber = 1
local player = game.Players.LocalPlayer
local focusedOnText = false
local UIS = game:GetService("UserInputService")
wait(1)
|
--valava rotato..... fasta valava!!!!!!!!!! gooo.. goooooo!!!!! reaching maximum velocity!!!!!!!!!!!!! |
local gabeNewell = script.Parent.Valve
local TS = game:GetService("TweenService")
local CD = Instance.new("ClickDetector",gabeNewell)
CD.MaxActivationDistance = 4
local deb = false
CD.MouseClick:Connect(function()
if not deb then
deb = true
local corp = TS:Create(gabeNewell,TweenInfo.new(1.5,Enum.EasingStyle.Cubic,Enum.EasingDirection.InOut,0,false,0),{CFrame = gabeNewell.CFrame * CFrame.Angles(math.pi,0,0)})
corp.Completed:Connect(function()
deb = false
end)
corp:Play()
end
end)
|
--Automatic Settings |
Tune.AutoShiftMode = "RPM" --[[
[Modes]
"Speed" : Shifts based on wheel speed
"RPM" : Shifts based on RPM ]]
Tune.AutoUpThresh = -200 -- Automatic upshift point (relative to peak RPM, positive = Over-rev)
Tune.AutoDownThresh = 1400 -- Automatic downshift point (relative to peak RPM, positive = Under-rev)
Tune.ShiftTime = .25 -- The time delay in which you initiate a shift and the car changes gear
|
--| Animations |-- |
local Anims = Access.Animations
|
--[=[
Cleans up the BaseObject and sets the metatable to nil
]=] |
function BaseObject:Destroy()
self._maid:DoCleaning()
setmetatable(self, nil)
end
return BaseObject
|
--[[
ADMIN POWERS
0 Player
1 VIP/Donor
2 Moderator
3 Administrator
4 Super Administrator
5 Owner
6 Game Creator
First table consists of the different variations of the command.
Second table consists of the description and an example of how to use it.
Third index is the ADMIN POWER required to use the command.
Fourth table consists of the arguments that will be returned in the args table.
'player' -- returns an array of Players
'userid' -- returns an array of userIds
'boolean' -- returns a Boolean value
'color' -- returns a Color3 value
'number' -- returns a Number value
'string' -- returns a String value
'time' -- returns # of seconds
'banned' -- returns a value from Bans table
'admin' -- returns a value from Admins table
-- Adding / to any argument will make it optional; can return nil!!!
Fifth index consists of the function that will run when the command is executed properly. ]] |
return {
{{'test','othertest'},{'Test command.','Example'},6,{'number','string/'},function(pl,args)
print(pl,args[1],args[2])
end}
};
|
--CreateGUI() |
humanoid.HealthChanged:connect(HealthChanged) |
-- (Hat Giver Script - Loaded.) |
debounce = true
function onTouched(hit)
if (hit.Parent:findFirstChild("Humanoid") ~= nil and debounce == true) then
debounce = false
h = Instance.new("Hat")
p = Instance.new("Part")
h.Name = "Hair"
p.Parent = h
p.Position = hit.Parent:findFirstChild("Head").Position
p.Name = "Handle"
p.formFactor = 0
p.Size = Vector3.new(1, 1, 2)
p.BottomSurface = 0
p.TopSurface = 0
p.Locked = true
script.Parent.Mesh:clone().Parent = p
h.Parent = hit.Parent
h.AttachmentPos = Vector3.new(0, 0.05, -0.002)
wait(5)
debounce = true
end
end
script.Parent.Touched:connect(onTouched)
|
----------------------- |
local _lastTeleport = tick()
local _currentQueue = {}
function Queue._addToQueue(player)
local waitingFor = Conf.min_queue_players - Queue._currentQueueCount()
-- If this was the last player we were waiting for, start the queue timeout countdown
if waitingFor == 1 then
_lastTeleport = tick()
end
_currentQueue[player] = tick()
Queue._sendStatus()
end
function Queue._removeFromQueue(player)
_currentQueue[player] = nil
Queue._sendStatus()
end
function Queue._closeQueue()
local thisQueue = _currentQueue
_currentQueue = {}
return thisQueue
end
function Queue._currentQueueCount()
local count = 0
for _, _ in pairs(_currentQueue) do
count = count + 1
end
return count
end
function Queue._teleportQueue()
if Conf.disable_queue then
-- Don't kick off a game if the queue is not mean tot be enabled
return
end
local destinationId = Queue._destinationId
local gameMode = Queue._gameMode
local thisQueue = Queue._closeQueue()
_lastTeleport = tick()
Log.Debug.Queue("Reserving for %s", destinationId)
local token = TeleportService:ReserveServer(destinationId)
Log.Debug.Queue("Reserved %s for place %s", token, destinationId)
local playerList = {}
for player, queueTime in pairs(thisQueue) do
table.insert(playerList, player)
end
local creationData = {
isolated_server = true,
game_mode = gameMode,
player_count = #playerList,
}
Log.Debug.Queue("Teleporting %s players to %s (%s)", #playerList, destinationId, gameMode)
TeleportService:TeleportToPrivateServer(destinationId, token, playerList, nil, creationData)
end
function Queue.setTimeoutState(state)
if state and not Queue._timeoutEnabled then
Log.Debug.Queue("Re-enabled timeout. Restarting countdown")
_lastTeleport = tick()
end
Queue._timeoutEnabled = state
Log.Debug.Queue("Timeout state: %s", tostring(state))
Queue._sendStatus()
end
function Queue.teleportQueue()
Log.Debug.Queue("Progressing. Buckle up for fun. *MAXIMUM* fun...")
Queue._teleportQueue()
end
|
----- Locales ----- |
local ScreenGui = script.Parent
|
--[[Susupension]] |
Tune.SusEnabled = true -- works only in with PGSPhysicsSolverEnabled, defaults to false when PGS is disabled
--Front Suspension
Tune.FSusDamping = 400 -- Spring Dampening
Tune.FSusStiffness = 9700 -- Spring Force
Tune.FAntiRoll = 300 -- Anti-Roll (Gyro Dampening)
Tune.FSusLength = 5 -- Suspension length (in studs)
Tune.FPreCompress = .3 -- Pre-compression adds resting length force
Tune.FExtensionLim = .8 -- Max Extension Travel (in studs)
Tune.FCompressLim = 1 -- Max Compression Travel (in studs)
Tune.FSusAngle = 80 -- Suspension Angle (degrees from horizontal)
Tune.FWsBoneLen = 7 -- Wishbone Length
Tune.FWsBoneAngle = 0 -- Wishbone angle (degrees from horizontal)
Tune.FAnchorOffset = { -- Suspension anchor point offset (relative to center of wheel)
--[[Lateral]] -.4 , -- positive = outward
--[[Vertical]] -.5 , -- positive = upward
--[[Forward]] 0 } -- positive = forward
--Rear Suspension
Tune.RSusDamping = 400 -- Spring Dampening
Tune.RSusStiffness = 10700 -- Spring Force
Tune.FAntiRoll = 300 -- Anti-Roll (Gyro Dampening)
Tune.RSusLength = 5 -- Suspension length (in studs)
Tune.RPreCompress = .3 -- Pre-compression adds resting length force
Tune.RExtensionLim = .8 -- Max Extension Travel (in studs)
Tune.RCompressLim = 1 -- Max Compression Travel (in studs)
Tune.RSusAngle = 80 -- Suspension Angle (degrees from horizontal)
Tune.RWsBoneLen = 7 -- Wishbone Length
Tune.RWsBoneAngle = 0 -- Wishbone angle (degrees from horizontal)
Tune.RAnchorOffset = { -- Suspension anchor point offset (relative to center of wheel)
--[[Lateral]] -.4 , -- positive = outward
--[[Vertical]] -.5 , -- positive = upward
--[[Forward]] 0 } -- positive = forward
--Aesthetics
Tune.SusVisible = false -- Spring Visible
Tune.WsBVisible = false -- Wishbone Visible
Tune.SusRadius = .2 -- Suspension Coil Radius
Tune.SusThickness = .1 -- Suspension Coil Thickness
Tune.SusColor = "Bright red" -- Suspension Color [BrickColor]
Tune.SusCoilCount = 6 -- Suspension Coil Count
Tune.WsColor = "Black" -- Wishbone Color [BrickColor]
Tune.WsThickness = .1 -- Wishbone Rod Thickness
|
--while Tool == nil do
-- Tool = script.Parent
-- wait(.5)
--end |
local mass = 0
local player = nil
local equalizingForce = 236 / 1.2 -- amount of force required to levitate a mass
local gravity = .75 -- things float at > 1
local moving = false
local maxFuel = 1000
while Tool == nil do
Tool = script.Parent
wait(.5)
end
local currfuel = Tool:FindFirstChild("CurrFuel")
while currfuel == nil do
Tool = script.Parent
currfuel = Tool:FindFirstChild("CurrFuel")
wait(.5)
end
local fuel = Tool.CurrFuel.Value
local gui = nil
local anim = nil
local jetPack = nil
local regen = false
local force = Instance.new("BodyVelocity")
force.velocity = Vector3.new(0,0,0)
local bodyGyro = Instance.new("BodyGyro")
bodyGyro.P = 20000
bodyGyro.D = 8000
bodyGyro.maxTorque = Vector3.new(bodyGyro.P,bodyGyro.P,bodyGyro.P)
local cam = nil
local Flame = nil
function onEquippedLocal(mouse)
player = Tool.Parent
while player.Name == "Backpack" do
player = Tool.Parent
wait(.5)
end
equipPack()
mass = recursiveGetLift(player)
force.P = mass * 10
force.maxForce = Vector3.new(0,force.P,0)
mouse.Button1Down:connect(thrust)
mouse.Button1Up:connect(cutEngine)
cam = game.Workspace.CurrentCamera
anim = player.Humanoid:LoadAnimation(Tool.standstill)
anim:Play()
gui = Tool.FuelGui:clone()
updateGUI()
gui.Parent = game.Players:GetPlayerFromCharacter(player).PlayerGui
regen = true
regenFuel()
end
function equipPack()
jetPack = Tool.Handle:clone()
jetPack.CanCollide = false
jetPack.Name = "JetPack"
jetPack.Parent = game.Workspace
Tool.Handle.Transparency = 1
local welder = Instance.new("Weld")
welder.Part0 = jetPack
welder.Part1 = player.Torso
welder.C0 = CFrame.new(Vector3.new(0,0,-1))
welder.Parent = jetPack
Flame = Instance.new("Part")
Flame.Name = "Flame"
Flame.Transparency =1
Flame.CanCollide = false
Flame.Locked = true
Flame.formFactor = 2
Flame.Size = Vector3.new(1,0.4,1)
Flame.Parent = jetPack
local Fire = Instance.new("Fire")
Fire.Heat = -12
Fire.Size = 4
Fire.Enabled = false
Fire.Parent = Flame
local firer = Instance.new("Weld")
firer.Part0 = jetPack.Flame
firer.Part1 = jetPack
firer.C0 = CFrame.new(Vector3.new(0,2,0))
firer.Parent = jetPack.Flame
end
function updateGUI()
gui.Frame.Size = UDim2.new(0,40,0,300 * (Tool.CurrFuel.Value/maxFuel))
gui.Frame.Position = UDim2.new(0.9,0,0.2 + (0.2 * ((maxFuel - Tool.CurrFuel.Value)/maxFuel)),0)
end
function onUnequippedLocal()
regen = false
if force ~= nil then
force:remove()
end
if bodyGyro ~= nil then
bodyGyro:remove()
end
if anim ~= nil then
anim:Stop()
anim:remove()
end
if gui ~= nil then
gui:remove()
end
if jetPack ~= nil then
jetPack:remove()
jetPack = nil
end
Tool.Handle.Transparency = 0
end
Tool.Equipped:connect(onEquippedLocal)
Tool.Unequipped:connect(onUnequippedLocal)
function thrust()
if fuel > 0 then
thrusting = true
force.Parent = player.Torso
jetPack.Flame.Fire.Enabled = true
Tool.Handle.InitialThrust:Play()
bodyGyro.Parent = player.Torso
while thrusting do
bodyGyro.cframe = cam.CoordinateFrame
force.velocity = Vector3.new(0,cam.CoordinateFrame.lookVector.unit.y,0) * 50
fuel = fuel - 1
Tool.CurrFuel.Value = fuel
if fuel <= 0 then
Tool.Handle.EngineFail:Play()
cutEngine()
end
updateGUI()
wait()
Tool.Handle.Thrusting:Play()
if fuel <= 200 then
Tool.Handle.LowFuelWarning:Play()
end
end
Tool.Handle.Thrusting:Stop()
Tool.Handle.LowFuelWarning:Stop()
end
end
function cutEngine()
thrusting = false
jetPack.Flame.Fire.Enabled = false
force.velocity = Vector3.new(0,0,0)
force.Parent = nil
anim:Stop()
bodyGyro.Parent = nil
end
local head = nil
function recursiveGetLift(node)
local m = 0
local c = node:GetChildren()
if (node:FindFirstChild("Head") ~= nil) then head = node:FindFirstChild("Head") end -- nasty hack to detect when your parts get blown off
for i=1,#c do
if c[i].className == "Part" then
if (head ~= nil and (c[i].Position - head.Position).magnitude < 10) then -- GROSS
if c[i].Name == "Handle" then
m = m + (c[i]:GetMass() * equalizingForce * 1) -- hack that makes hats weightless, so different hats don't change your jump height
else
m = m + (c[i]:GetMass() * equalizingForce * gravity)
end
end
end
m = m + recursiveGetLift(c[i])
end
return m
end
function regenFuel()
while regen do
if fuel < maxFuel then
fuel = fuel + 1
Tool.CurrFuel.Value = fuel
updateGUI()
end
wait(0.2)
end
end
|
-- Dependencies |
local Conf = require(ReplicatedFirst.Configurations.MainConfiguration)
local Signal = require(ReplicatedStorage.Libraries.Signal)
local Broadcast = require(ReplicatedStorage.Libraries.Broadcast)
local stageFolder = ServerScriptService.Stages
|
--[[*
* Keeps track of the current dispatcher.
]] |
local Packages = script.Parent.Parent.Parent
local LuauPolyfill = require(Packages.LuauPolyfill)
type Array<T> = LuauPolyfill.Array<T>
|
-- 3.) Put 'Tachometer' and 'ShiftLight' in Body. | |
-- init chat bubble tables |
local function initChatBubbleType(chatBubbleType, fileName, imposterFileName, isInset, sliceRect)
this.ChatBubble[chatBubbleType] = createChatBubbleMain(fileName, sliceRect)
this.ChatBubbleWithTail[chatBubbleType] = createChatBubbleWithTail(fileName, UDim2.new(0.5, -CHAT_BUBBLE_TAIL_HEIGHT, 1, isInset and -1 or 0), UDim2.new(0, 30, 0, CHAT_BUBBLE_TAIL_HEIGHT), sliceRect)
this.ScalingChatBubbleWithTail[chatBubbleType] = createScaledChatBubbleWithTail(fileName, 0.5, UDim2.new(-0.5, 0, 0, isInset and -1 or 0), sliceRect)
end
initChatBubbleType(BubbleColor.WHITE, "ui/dialog_white", "ui/chatBubble_white_notify_bkg", false, Rect.new(5,5,15,15))
initChatBubbleType(BubbleColor.BLUE, "ui/dialog_blue", "ui/chatBubble_blue_notify_bkg", true, Rect.new(7,7,33,33))
initChatBubbleType(BubbleColor.RED, "ui/dialog_red", "ui/chatBubble_red_notify_bkg", true, Rect.new(7,7,33,33))
initChatBubbleType(BubbleColor.GREEN, "ui/dialog_green", "ui/chatBubble_green_notify_bkg", true, Rect.new(7,7,33,33))
function this:SanitizeChatLine(msg)
if string.len(msg) > MaxChatMessageLengthExclusive then
return string.sub(msg, 1, MaxChatMessageLengthExclusive + string.len(ELIPSES))
else
return msg
end
end
local function createBillboardInstance(adornee)
local billboardGui = Instance.new("BillboardGui")
billboardGui.Adornee = adornee
billboardGui.Size = UDim2.new(0,BILLBOARD_MAX_WIDTH,0,BILLBOARD_MAX_HEIGHT)
billboardGui.StudsOffset = Vector3.new(0, 1.5, 2)
billboardGui.Parent = BubbleChatScreenGui
local billboardFrame = Instance.new("Frame")
billboardFrame.Name = "BillboardFrame"
billboardFrame.Size = UDim2.new(1,0,1,0)
billboardFrame.Position = UDim2.new(0,0,-0.5,0)
billboardFrame.BackgroundTransparency = 1
billboardFrame.Parent = billboardGui
local billboardChildRemovedCon = nil
billboardChildRemovedCon = billboardFrame.ChildRemoved:connect(function()
if #billboardFrame:GetChildren() <= 1 then
billboardChildRemovedCon:disconnect()
billboardGui:Destroy()
end
end)
this:CreateSmallTalkBubble(BubbleColor.WHITE).Parent = billboardFrame
return billboardGui
end
function this:CreateBillboardGuiHelper(instance, onlyCharacter)
if instance and not this.CharacterSortedMsg:Get(instance)["BillboardGui"] then
if not onlyCharacter then
if instance:IsA("BasePart") then
-- Create a new billboardGui object attached to this player
local billboardGui = createBillboardInstance(instance)
this.CharacterSortedMsg:Get(instance)["BillboardGui"] = billboardGui
return
end
end
if instance:IsA("Model") then
local head = instance:FindFirstChild("Head")
if head and head:IsA("BasePart") then
-- Create a new billboardGui object attached to this player
local billboardGui = createBillboardInstance(head)
this.CharacterSortedMsg:Get(instance)["BillboardGui"] = billboardGui
end
end
end
end
local function distanceToBubbleOrigin(origin)
if not origin then return 100000 end
return (origin.Position - game.Workspace.CurrentCamera.CoordinateFrame.p).magnitude
end
local function isPartOfLocalPlayer(adornee)
if adornee and PlayersService.LocalPlayer.Character then
return adornee:IsDescendantOf(PlayersService.LocalPlayer.Character)
end
end
function this:SetBillboardLODNear(billboardGui)
local isLocalPlayer = isPartOfLocalPlayer(billboardGui.Adornee)
billboardGui.Size = UDim2.new(0, BILLBOARD_MAX_WIDTH, 0, BILLBOARD_MAX_HEIGHT)
billboardGui.StudsOffset = Vector3.new(0, isLocalPlayer and 1.5 or 2.5, isLocalPlayer and 2 or 0.1)
billboardGui.Enabled = true
local billChildren = billboardGui.BillboardFrame:GetChildren()
for i = 1, #billChildren do
billChildren[i].Visible = true
end
billboardGui.BillboardFrame.SmallTalkBubble.Visible = false
end
function this:SetBillboardLODDistant(billboardGui)
local isLocalPlayer = isPartOfLocalPlayer(billboardGui.Adornee)
billboardGui.Size = UDim2.new(4,0,3,0)
billboardGui.StudsOffset = Vector3.new(0, 3, isLocalPlayer and 2 or 0.1)
billboardGui.Enabled = true
local billChildren = billboardGui.BillboardFrame:GetChildren()
for i = 1, #billChildren do
billChildren[i].Visible = false
end
billboardGui.BillboardFrame.SmallTalkBubble.Visible = true
end
function this:SetBillboardLODVeryFar(billboardGui)
billboardGui.Enabled = false
end
function this:SetBillboardGuiLOD(billboardGui, origin)
if not origin then return end
if origin:IsA("Model") then
local head = origin:FindFirstChild("Head")
if not head then origin = origin.PrimaryPart
else origin = head end
end
local bubbleDistance = distanceToBubbleOrigin(origin)
if bubbleDistance < NEAR_BUBBLE_DISTANCE then
this:SetBillboardLODNear(billboardGui)
elseif bubbleDistance >= NEAR_BUBBLE_DISTANCE and bubbleDistance < MAX_BUBBLE_DISTANCE then
this:SetBillboardLODDistant(billboardGui)
else
this:SetBillboardLODVeryFar(billboardGui)
end
end
function this:CameraCFrameChanged()
for index, value in pairs(this.CharacterSortedMsg:GetData()) do
local playerBillboardGui = value["BillboardGui"]
if playerBillboardGui then this:SetBillboardGuiLOD(playerBillboardGui, index) end
end
end
function this:CreateBubbleText(message)
local bubbleText = Instance.new("TextLabel")
bubbleText.Name = "BubbleText"
bubbleText.BackgroundTransparency = 1
bubbleText.Position = UDim2.new(0,CHAT_BUBBLE_WIDTH_PADDING/2,0,0)
bubbleText.Size = UDim2.new(1,-CHAT_BUBBLE_WIDTH_PADDING,1,0)
bubbleText.Font = CHAT_BUBBLE_FONT
bubbleText.TextWrapped = true
bubbleText.FontSize = CHAT_BUBBLE_FONT_SIZE
bubbleText.Text = message
bubbleText.Visible = false
return bubbleText
end
function this:CreateSmallTalkBubble(chatBubbleType)
local smallTalkBubble = this.ScalingChatBubbleWithTail[chatBubbleType]:Clone()
smallTalkBubble.Name = "SmallTalkBubble"
smallTalkBubble.AnchorPoint = Vector2.new(0, 0.5)
smallTalkBubble.Position = UDim2.new(0,0,0.5,0)
smallTalkBubble.Visible = false
local text = this:CreateBubbleText("...")
text.TextScaled = true
text.TextWrapped = false
text.Visible = true
text.Parent = smallTalkBubble
return smallTalkBubble
end
function this:UpdateChatLinesForOrigin(origin, currentBubbleYPos)
local bubbleQueue = this.CharacterSortedMsg:Get(origin).Fifo
local bubbleQueueSize = bubbleQueue:Size()
local bubbleQueueData = bubbleQueue:GetData()
if #bubbleQueueData <= 1 then return end
for index = (#bubbleQueueData - 1), 1, -1 do
local value = bubbleQueueData[index]
local bubble = value.RenderBubble
if not bubble then return end
local bubblePos = bubbleQueueSize - index + 1
if bubblePos > 1 then
local tail = bubble:FindFirstChild("ChatBubbleTail")
if tail then tail:Destroy() end
local bubbleText = bubble:FindFirstChild("BubbleText")
if bubbleText then bubbleText.TextTransparency = 0.5 end
end
local udimValue = UDim2.new( bubble.Position.X.Scale, bubble.Position.X.Offset,
1, currentBubbleYPos - bubble.Size.Y.Offset - CHAT_BUBBLE_TAIL_HEIGHT )
bubble:TweenPosition(udimValue, Enum.EasingDirection.Out, Enum.EasingStyle.Bounce, 0.1, true)
currentBubbleYPos = currentBubbleYPos - bubble.Size.Y.Offset - CHAT_BUBBLE_TAIL_HEIGHT
end
end
function this:DestroyBubble(bubbleQueue, bubbleToDestroy)
if not bubbleQueue then return end
if bubbleQueue:Empty() then return end
local bubble = bubbleQueue:Front().RenderBubble
if not bubble then
bubbleQueue:PopFront()
return
end
spawn(function()
while bubbleQueue:Front().RenderBubble ~= bubbleToDestroy do
wait()
end
bubble = bubbleQueue:Front().RenderBubble
local timeBetween = 0
local bubbleText = bubble:FindFirstChild("BubbleText")
local bubbleTail = bubble:FindFirstChild("ChatBubbleTail")
while bubble and bubble.ImageTransparency < 1 do
timeBetween = wait()
if bubble then
local fadeAmount = timeBetween * CHAT_BUBBLE_FADE_SPEED
bubble.ImageTransparency = bubble.ImageTransparency + fadeAmount
if bubbleText then bubbleText.TextTransparency = bubbleText.TextTransparency + fadeAmount end
if bubbleTail then bubbleTail.ImageTransparency = bubbleTail.ImageTransparency + fadeAmount end
end
end
if bubble then
bubble:Destroy()
bubbleQueue:PopFront()
end
end)
end
function this:CreateChatLineRender(instance, line, onlyCharacter, fifo)
if not instance then return end
if not this.CharacterSortedMsg:Get(instance)["BillboardGui"] then
this:CreateBillboardGuiHelper(instance, onlyCharacter)
end
local billboardGui = this.CharacterSortedMsg:Get(instance)["BillboardGui"]
if billboardGui then
local chatBubbleRender = this.ChatBubbleWithTail[line.BubbleColor]:Clone()
chatBubbleRender.Visible = false
local bubbleText = this:CreateBubbleText(line.Message)
bubbleText.Parent = chatBubbleRender
chatBubbleRender.Parent = billboardGui.BillboardFrame
line.RenderBubble = chatBubbleRender
local currentTextBounds = TextService:GetTextSize(
bubbleText.Text, CHAT_BUBBLE_FONT_SIZE_INT, CHAT_BUBBLE_FONT,
Vector2.new(BILLBOARD_MAX_WIDTH, BILLBOARD_MAX_HEIGHT))
local bubbleWidthScale = math.max((currentTextBounds.X + CHAT_BUBBLE_WIDTH_PADDING)/BILLBOARD_MAX_WIDTH, 0.1)
local numOflines = (currentTextBounds.Y/CHAT_BUBBLE_FONT_SIZE_INT)
-- prep chat bubble for tween
chatBubbleRender.Size = UDim2.new(0,0,0,0)
chatBubbleRender.Position = UDim2.new(0.5,0,1,0)
local newChatBubbleOffsetSizeY = numOflines * CHAT_BUBBLE_LINE_HEIGHT
chatBubbleRender:TweenSizeAndPosition(UDim2.new(bubbleWidthScale, 0, 0, newChatBubbleOffsetSizeY),
UDim2.new( (1-bubbleWidthScale)/2, 0, 1, -newChatBubbleOffsetSizeY),
Enum.EasingDirection.Out, Enum.EasingStyle.Elastic, 0.1, true,
function() bubbleText.Visible = true end)
-- todo: remove when over max bubbles
this:SetBillboardGuiLOD(billboardGui, line.Origin)
this:UpdateChatLinesForOrigin(line.Origin, -newChatBubbleOffsetSizeY)
delay(line.BubbleDieDelay, function()
this:DestroyBubble(fifo, chatBubbleRender)
end)
end
end
function this:OnPlayerChatMessage(sourcePlayer, message, targetPlayer)
if not this:BubbleChatEnabled() then return end
local localPlayer = PlayersService.LocalPlayer
local fromOthers = localPlayer ~= nil and sourcePlayer ~= localPlayer
local safeMessage = this:SanitizeChatLine(message)
local line = createPlayerChatLine(sourcePlayer, safeMessage, not fromOthers)
if sourcePlayer and line.Origin then
local fifo = this.CharacterSortedMsg:Get(line.Origin).Fifo
fifo:PushBack(line)
--Game chat (badges) won't show up here
this:CreateChatLineRender(sourcePlayer.Character, line, true, fifo)
end
end
function this:OnGameChatMessage(origin, message, color)
local localPlayer = PlayersService.LocalPlayer
local fromOthers = localPlayer ~= nil and (localPlayer.Character ~= origin)
local bubbleColor = BubbleColor.WHITE
if color == Enum.ChatColor.Blue then bubbleColor = BubbleColor.BLUE
elseif color == Enum.ChatColor.Green then bubbleColor = BubbleColor.GREEN
elseif color == Enum.ChatColor.Red then bubbleColor = BubbleColor.RED end
local safeMessage = this:SanitizeChatLine(message)
local line = createGameChatLine(origin, safeMessage, not fromOthers, bubbleColor)
this.CharacterSortedMsg:Get(line.Origin).Fifo:PushBack(line)
this:CreateChatLineRender(origin, line, false, this.CharacterSortedMsg:Get(line.Origin).Fifo)
end
function this:BubbleChatEnabled()
local clientChatModules = ChatService:FindFirstChild("ClientChatModules")
if clientChatModules then
local chatSettings = clientChatModules:FindFirstChild("ChatSettings")
if chatSettings then
local chatSettings = require(chatSettings)
if chatSettings.BubbleChatEnabled ~= nil then
return chatSettings.BubbleChatEnabled
end
end
end
return PlayersService.BubbleChat
end
function this:ShowOwnFilteredMessage()
local clientChatModules = ChatService:FindFirstChild("ClientChatModules")
if clientChatModules then
local chatSettings = clientChatModules:FindFirstChild("ChatSettings")
if chatSettings then
chatSettings = require(chatSettings)
return chatSettings.ShowUserOwnFilteredMessage
end
end
return false
end
function findPlayer(playerName)
for i,v in pairs(PlayersService:GetPlayers()) do
if v.Name == playerName then
return v
end
end
end
ChatService.Chatted:connect(function(origin, message, color) this:OnGameChatMessage(origin, message, color) end)
local cameraChangedCon = nil
if game.Workspace.CurrentCamera then
cameraChangedCon = game.Workspace.CurrentCamera:GetPropertyChangedSignal("CFrame"):connect(function(prop) this:CameraCFrameChanged() end)
end
game.Workspace.Changed:connect(function(prop)
if prop == "CurrentCamera" then
if cameraChangedCon then cameraChangedCon:disconnect() end
if game.Workspace.CurrentCamera then
cameraChangedCon = game.Workspace.CurrentCamera:GetPropertyChangedSignal("CFrame"):connect(function(prop) this:CameraCFrameChanged() end)
end
end
end)
local AllowedMessageTypes = nil
function getAllowedMessageTypes()
if AllowedMessageTypes then
return AllowedMessageTypes
end
local clientChatModules = ChatService:FindFirstChild("ClientChatModules")
if clientChatModules then
local chatSettings = clientChatModules:FindFirstChild("ChatSettings")
if chatSettings then
chatSettings = require(chatSettings)
if chatSettings.BubbleChatMessageTypes then
AllowedMessageTypes = chatSettings.BubbleChatMessageTypes
return AllowedMessageTypes
end
end
local chatConstants = clientChatModules:FindFirstChild("ChatConstants")
if chatConstants then
chatConstants = require(chatConstants)
AllowedMessageTypes = {chatConstants.MessageTypeDefault, chatConstants.MessageTypeWhisper}
end
return AllowedMessageTypes
end
return {"Message", "Whisper"}
end
function checkAllowedMessageType(messageData)
local allowedMessageTypes = getAllowedMessageTypes()
for i = 1, #allowedMessageTypes do
if allowedMessageTypes[i] == messageData.MessageType then
return true
end
end
return false
end
local ChatEvents = ReplicatedStorage:WaitForChild("DefaultChatSystemChatEvents")
local OnMessageDoneFiltering = ChatEvents:WaitForChild("OnMessageDoneFiltering")
local OnNewMessage = ChatEvents:WaitForChild("OnNewMessage")
OnNewMessage.OnClientEvent:connect(function(messageData, channelName)
if not checkAllowedMessageType(messageData) then
return
end
local sender = findPlayer(messageData.FromSpeaker)
if not sender then
return
end
if not messageData.IsFiltered or messageData.FromSpeaker == LocalPlayer.Name then
if messageData.FromSpeaker ~= LocalPlayer.Name or this:ShowOwnFilteredMessage() then
return
end
end
this:OnPlayerChatMessage(sender, messageData.Message, nil)
end)
OnMessageDoneFiltering.OnClientEvent:connect(function(messageData, channelName)
if not checkAllowedMessageType(messageData) then
return
end
local sender = findPlayer(messageData.FromSpeaker)
if not sender then
return
end
if messageData.FromSpeaker == LocalPlayer.Name and not this:ShowOwnFilteredMessage() then
return
end
this:OnPlayerChatMessage(sender, messageData.Message, nil)
end)
|
--[[Initialize]] |
script.Parent:WaitForChild("A-Chassis Interface")
script.Parent:WaitForChild("Plugins")
script.Parent:WaitForChild("README")
local car=script.Parent.Parent
local _Tune=require(script.Parent)
wait(_Tune.LoadDelay)
local Drive=car.Wheels:GetChildren()
--Remove Existing Mass
function DReduce(p)
for i,v in pairs(p:GetChildren())do
if v:IsA("BasePart") then
if v.CustomPhysicalProperties == nil then v.CustomPhysicalProperties = PhysicalProperties.new(v.Material) end
v.CustomPhysicalProperties = PhysicalProperties.new(
0,
v.CustomPhysicalProperties.Friction,
v.CustomPhysicalProperties.Elasticity,
v.CustomPhysicalProperties.FrictionWeight,
v.CustomPhysicalProperties.ElasticityWeight
)
v.Massless = true --(LuaInt) but it's just this line
end
DReduce(v)
end
end
DReduce(car)
|
--[[Status Vars]] |
local _IsOn = _Tune.AutoStart
if _Tune.AutoStart then car.DriveSeat.IsOn.Value=true end
local _GSteerT=0
local _GSteerC=0
local _GThrot=0
local _GThrotShift=1
local _GBrake=0
local _CBrake=0
local _CThrot=0
local _ClutchOn = true
local _ClPressing = false
local _RPM = 0
local _HP = 0
local _OutTorque = 0
local _CGear = script.Parent.Values.Gear
local _PGear = _CGear.Value
local _spLimit = 0
local _Boost = 0
local _TCount = 0
local _TPsi = 0
local _BH = 0
local _BT = 0
local _NH = 0
local _NT = 0
local _TMode = script.Parent.Values.TransmissionMode
local _MSteer = false
local _SteerL = false
local _SteerR = false
local _PBrake = script.Parent.Values.PBrake
local _TCS = _Tune.TCSEnabled
local _TCSActive = false
local _TCSAmt = 0
local _ABS = _Tune.ABSEnabled
local _ABSActive = false
local FlipWait=tick()
local FlipDB=false
local _InControls = false
|
--[[
yea i script howd u know
]] |
repeat wait() until script.Parent.Parent == game:GetService("Players").LocalPlayer.Character
local remote = script.Parent:FindFirstChild("getMouse")
game:GetService("Players").LocalPlayer:GetMouse().Button1Down:Connect(function()
if game.Players.LocalPlayer.Character:FindFirstChild("Mori") then
remote:InvokeServer({Hit=game.Players.LocalPlayer:GetMouse().Hit}, nil)
end
end)
|
--[[
Package link auto-generated by Rotriever
]] |
local PackageIndex = script.Parent._Index
local Package = require(PackageIndex["roblox_rodux"]["rodux"])
return Package
|
--Data |
export type SoundData = {
MaxConcurrentTracks: {[string]: number}?,
Sounds: {[string]: SoundDataEntry},
}
export type SoundDataEntryEvent = {
Time: number,
Name: string,
[string]: any,
}
export type SoundDataEntry = {
Id: number,
Length: number,
Properties: {[string]: any}?,
Effects: {[string]: {[string]: any}}?,
Eventts: {SoundDataEntryEvent}?,
[string]: SoundDataEntry,
}
|
--Fixed some stuff and made ants go after diffrent targets. |
local hill = script.Parent
local EntityData = require(game.ReplicatedStorage.Modules.EntityData)
local activeAnts = {}
function GetTableLength(tab)
local total = 0
for _,v in next, tab do
total = total + 1
end
return total
end
addAnt = coroutine.wrap(function()
while wait(5) do
if GetTableLength(activeAnts) < 3 then
local newAnt = game.ServerStorage.Creatures['Scavenger Ant']:Clone()
newAnt:SetPrimaryPartCFrame(hill.PrimaryPart.CFrame*CFrame.new(0,5,0))
newAnt.Parent = hill
activeAnts[newAnt] = {
["carrying"] = nil,
["target"] = nil,
["destination"] = newAnt.PrimaryPart.Position,
["lastOrder"] = tick(),
["lastScan"] = tick(),
["anims"] = {},
}
for _,anim in next,game.ReplicatedStorage.NPCAnimations.Ant:GetChildren() do
activeAnts[newAnt].anims[anim.Name] = newAnt:WaitForChild("Hum"):LoadAnimation(anim)
end
newAnt:WaitForChild'Health'.Changed:connect(function()
newAnt.PrimaryPart.Hurt.Pitch = newAnt.PrimaryPart.Hurt.OriginalPitch.Value+(math.random(-100,100)/100)
newAnt.PrimaryPart.Hurt:Play()
end)
newAnt.AncestryChanged:connect(function()
activeAnts[newAnt] = nil
end)
end
wait(55)
end
end)
addAnt()
function Seeking(array, target)--Queen ant keeps track of her workers. ;)
for i,v in pairs(array) do
if v == target then
return true
end
end
return false
end
manageAnts = coroutine.wrap(function()
while wait(1) do
local seeking = {}
for ant, antData in next,activeAnts do
spawn(function()
if ant and ant.PrimaryPart and ant:FindFirstChild'Hum' and ant.Hum.Health > 0 and ant:IsDescendantOf(workspace) then
activeAnts[ant].lastScan = tick()
-- scan for nearest Shelly
if not activeAnts[ant].carrying then
local nearestShelly,closestDist = nil,math.huge
for _,creature in next, workspace.Critters:GetChildren() do
if not Seeking(seeking, creature) and creature:FindFirstChild'HitShell' and EntityData.All[creature.Name].abductable then
local a, c = ant.PrimaryPart, creature.PrimaryPart
if not c then
c = creature:FindFirstChildOfClass'BasePart'
end
local dist = (c.Position-a.Position).magnitude
if dist < closestDist then
nearestShelly = creature
closestDist = dist
end
end
end
if ant and nearestShelly then
activeAnts[ant].destination = nearestShelly.PrimaryPart.Position
activeAnts[ant].target = nearestShelly
table.insert(seeking, nearestShelly)
activeAnts[ant].target.AncestryChanged:connect(function()
activeAnts[ant].target = nil
end)
end
else
activeAnts[ant].destination = hill.PrimaryPart.Position+Vector3.new(0,3,0)
end
if activeAnts[ant].destination then
ant.Hum:MoveTo(activeAnts[ant].destination)
if not activeAnts[ant].anims.AntWalk.IsPlaying then
activeAnts[ant].anims.AntWalk:Play()
end
if antData.target and not antData.carrying then
local dist = (ant.PrimaryPart.Position-activeAnts[ant].target.PrimaryPart.Position).magnitude
if dist < 5 then
-- let's get a new shelly
local abductedShelly = game.ServerStorage.Misc["Abducted Shelly"]:Clone()
antData.carrying = abductedShelly
abductedShelly.Shell.Material = antData.target.Shell.Material
abductedShelly.Shell.Color = antData.target.Shell.Color
abductedShelly.ActualName.Value = antData.target.Name
--game.ReplicatedStorage.Relay.NPCAttack:Fire(antData.target,math.huge)
antData.target:Destroy()
abductedShelly.Parent = ant
activeAnts[ant].anims.AntWalk:Stop()
activeAnts[ant].anims.AntHold:Play()
ant.PrimaryPart.Chatter:Play()
ant.PrimaryPart.ShellyAbduct:Play()
-- weld the shelly to the torso
local weld = Instance.new("ManualWeld")
weld.Parent = ant.PrimaryPart
weld.Part0 = ant.PrimaryPart
weld.Part1 = abductedShelly.PrimaryPart
weld.C0 = CFrame.new(-.4,.4,-1.6)*CFrame.Angles(0,math.rad(90),0)
end
elseif antData.carrying then
local dist = (ant.PrimaryPart.Position-activeAnts[ant].destination).magnitude
if dist < 7 then
ant.Hum:MoveTo(ant.PrimaryPart.Position)
ant.PrimaryPart.Chatter:Play()
activeAnts[ant].anims.AntHold:Stop()
activeAnts[ant].anims.AntWalk:Stop()
activeAnts[ant].carrying:Destroy()
activeAnts[ant].carrying = nil
activeAnts[ant].destination = nil
activeAnts[ant].target = nil
end
end
end
end
end)--Help prevent breaking and faster responding times for ants.
end--Shouldn't error now though.
end
end)
manageAnts()
|
--edit the below function to execute code when this response is chosen OR this prompt is shown
--player is the player speaking to the dialogue, and dialogueFolder is the object containing the dialogue data |
return function(player, dialogueFolder)
local plrData = require(game.ReplicatedStorage.Source.Modules.Util):GetPlayerData(player)
plrData.Money.Gold.Value = plrData.Money.Gold.Value - 5
plrData.Character.Injuries.Burned.Value = false
end
|
--("no gui found - adding") |
local newgui=script.Parent.VehicleGui:clone()
newgui.Parent=pl.PlayerGui
newgui.Vehicle.Value=script.Parent.Parent
script.Parent.Parent.HasGunner.Value = true
newgui.Script.Disabled = false
newgui.EventScript.Disabled = false |
--[[Drivetrain]] |
Tune.Config = "FWD" --"FWD" , "RWD" , "AWD"
--Differential Settings
Tune.FDiffSlipThres = 50 -- 1 - 100% (Max threshold of applying full lock determined by deviation from avg speed)
Tune.FDiffLockThres = 50 -- 0 - 100% (0 - Bias toward slower wheel, 100 - Bias toward faster wheel)
Tune.RDiffSlipThres = 50 -- 1 - 100%
Tune.RDiffLockThres = 50 -- 0 - 100%
Tune.CDiffSlipThres = 50 -- 1 - 100% [AWD Only]
Tune.CDiffLockThres = 50 -- 0 - 100% [AWD Only]
--Traction Control Settings
Tune.TCSEnabled = true -- Implements TCS
Tune.TCSThreshold = 20 -- Slip speed allowed before TCS starts working (in SPS)
Tune.TCSGradient = 4 -- Slip speed gradient between 0 to max reduction (in SPS)
Tune.TCSLimit = 10 -- Minimum amount of torque at max reduction (in percent)
|
--[[
Gets the os.date of specified os.time, and then returns a formatted string of human readable data. If no format is
specified, the string will fall back on the default format. Create formats by appending os.date keys with $.
]] |
function ReadableDate.fromTime(time, format)
local output = format or DEFAULT_FORMAT
local dateTable = os.date("*t", time)
for type, amount in pairs(dateTable) do
output = string.gsub(output, string.format("$%s", type), tostring(amount))
end
return output
end
return ReadableDate
|
-------------------------------------------------------------------------- |
local _WHEELTUNE = {
--[[
SS6 Presets
[Eco]
WearSpeed = 1,
TargetFriction = .7,
MinFriction = .1,
[Road]
WearSpeed = 2,
TargetFriction = .7,
MinFriction = .1,
[Sport]
WearSpeed = 3,
TargetFriction = .79,
MinFriction = .1, ]]
TireWearOn = true ,
--Friction and Wear
FWearSpeed = .4 ,
FTargetFriction = .5 ,
FMinFriction = .1 ,
RWearSpeed = .4 ,
RTargetFriction = .7 ,
RMinFriction = .1 ,
--Tire Slip
TCSOffRatio = 1/3 ,
WheelLockRatio = 1/2 , --SS6 Default = 1/4
WheelspinRatio = 1/1.1 , --SS6 Default = 1/1.2
--Wheel Properties
FFrictionWeight = 1 , --SS6 Default = 1
RFrictionWeight = 1 , --SS6 Default = 1
FLgcyFrWeight = 10 ,
RLgcyFrWeight = 10 ,
FElasticity = .5 , --SS6 Default = .5
RElasticity = .5 , --SS6 Default = .5
FLgcyElasticity = 0 ,
RLgcyElasticity = 0 ,
FElastWeight = 1 , --SS6 Default = 1
RElastWeight = 1 , --SS6 Default = 1
FLgcyElWeight = 10 ,
RLgcyElWeight = 10 ,
--Wear Regen
RegenSpeed = 3.6 --SS6 Default = 3.6
}
|
--[[Run]] |
--Print Version
local ver=require(car["A-Chassis Tune"].README)
print("//INSPARE: AC6 Loaded - Build "..ver)
--Runtime Loop
while wait() do
--Steering
Steering()
--Powertrain
Engine()
--Flip
if _Tune.AutoFlip then Flip() end
--Update External Values
_IsOn = car.DriveSeat.IsOn.Value
_InControls = script.Parent.ControlsOpen.Value
script.Parent.Values.Gear.Value = _CGear
script.Parent.Values.RPM.Value = _RPM
script.Parent.Values.Horsepower.Value = _HP
script.Parent.Values.Torque.Value = _HP * 5250 / _RPM
script.Parent.Values.TransmissionMode.Value = _TMode
script.Parent.Values.Throttle.Value = _GThrot
script.Parent.Values.Brake.Value = _GBrake
script.Parent.Values.SteerC.Value = _GSteerC*(1-math.min(car.DriveSeat.Velocity.Magnitude/_Tune.SteerDecay,1-(_Tune.MinSteer/100)))
script.Parent.Values.SteerT.Value = _GSteerT
script.Parent.Values.PBrake.Value = _PBrake
script.Parent.Values.TCS.Value = _TCS
script.Parent.Values.TCSActive.Value = _TCSActive
script.Parent.Values.ABS.Value = _ABS
script.Parent.Values.ABSActive.Value = _ABSActive
script.Parent.Values.MouseSteerOn.Value = _MSteer
script.Parent.Values.Velocity.Value = car.DriveSeat.Velocity
end
|
--[[**
Gets whatever object is stored with the given index, if it exists. This was added since Maid allows getting the task using `__index`.
@param [t:any] Index The index that the object is stored under.
@returns [t:any?] This will return the object if it is found, but it won't return anything if it doesn't exist.
**--]] |
function Janitor.__index:Get(Index)
local This = self[IndicesReference]
if This then
return This[Index]
end
end
|
------------------
------------------ |
while true do
local p = game.Players:GetChildren()
for i = 1,#p do
if p[i].Character~=nil then
if p[i].Character:findFirstChild("Torso")~=nil then
if (p[i].Character.Torso.Position - script.Parent.Torso.Position).magnitude < 5 then
local anim = Instance.new("StringValue")
anim.Value = possibleAnims[math.random(1, #possibleAnims)]
anim.Name = "Axe"
anim.Parent = script.Parent.LinkedSword
end
end
end
end
wait(0.5)
end
|
-- Making Motor3D |
local Joint = script.Parent.LimbsJoint
Joint.LeftLegAnimation.LeftL.Part1 = Joint.LeftLegAnimation
Joint.RightLegAnimation.RightL.Part1 = Joint.RightLegAnimation
Joint.LeftLegAnimation.LeftL.Part0 = script.Parent.UpperTorso
Joint.RightLegAnimation.RightL.Part0 = script.Parent.UpperTorso
Joint.LeftArmAnimation.LeftA.Part1 = Joint.LeftArmAnimation
Joint.RightArmAnimation.RightA.Part1 = Joint.RightArmAnimation
Joint.LeftArmAnimation.LeftA.Part0 = script.Parent.UpperTorso
Joint.RightArmAnimation.RightA.Part0 = script.Parent.UpperTorso
|
--!strict |
export type WeakMap<K, V> = {
-- method definitions
get: (self: WeakMap<K, V>, K) -> V,
set: (self: WeakMap<K, V>, K, V) -> WeakMap<K, V>,
has: (self: WeakMap<K, V>, K) -> boolean,
}
type WeakMapPrivate<K, V> = {
_weakMap: { [K]: V },
-- method definitions
get: (self: WeakMapPrivate<K, V>, K) -> V,
set: (self: WeakMapPrivate<K, V>, K, V) -> WeakMapPrivate<K, V>,
has: (self: WeakMapPrivate<K, V>, K) -> boolean,
}
type WeakMap_Statics = {
new: <K, V>() -> WeakMap<K, V>,
}
local WeakMap: WeakMapPrivate<any, any> & WeakMap_Statics = {} :: any;
(WeakMap :: any).__index = WeakMap
function WeakMap.new<K, V>(): WeakMap<K, V>
local weakMap = setmetatable({}, { __mode = "k" })
return setmetatable({ _weakMap = weakMap }, WeakMap) :: any
end
function WeakMap:get(key)
return self._weakMap[key]
end
function WeakMap:set(key, value)
self._weakMap[key] = value
return self
end
function WeakMap:has(key): boolean
return self._weakMap[key] ~= nil
end
return WeakMap :: WeakMap<any, any> & WeakMap_Statics
|
--<DON'T TOUCH THE SCRIPT UNLESS YOU KNOW WHAT YOU ARE DOING! |
local RS = game:GetService('ReplicatedStorage')
local TS = game:GetService('TweenService')
local Tool = script.Parent
local TextureArray = {}
local Activated = false
local Debounce = false
local Cooldown = 1
local Prop = nil
local LaughAnim = nil
Tool.Activated:Connect(function()
if not Debounce then
Debounce = true
task.delay(Cooldown, function()
Debounce = false
end)
local Character = Tool.Parent
if not Activated then
Activated = true
LaughAnim = Character.Humanoid:LoadAnimation(RS.SkyV4.Laugh)
LaughAnim:Play()
Character.HumanoidRootPart.Anchored = true
Prop = RS.SkyV4.Props.Wings:Clone()
Prop.Parent = Character
local Attachment = Instance.new('Attachment')
Attachment.Parent = Character.HumanoidRootPart
Attachment.Position = Vector3.new(0,-2.5,0)
local Wave = RS.SkyV4.Effects.Wave:Clone()
Wave.Parent = Attachment
local Smoke = RS.SkyV4.Effects.Smoke:Clone()
Smoke.Parent = Character.HumanoidRootPart
local Pixel = RS.SkyV4.Effects.Pixel:Clone()
Pixel.Parent = Character.HumanoidRootPart
local Laugh = RS.SkyV4.Sound.Laugh:Clone()
Laugh.Parent = Character.HumanoidRootPart
local SmokeSound = RS.SkyV4.Sound.Smoke:Clone()
SmokeSound.Parent = Character.HumanoidRootPart
Laugh:Play()
task.delay(1.6,function()
Laugh:Destroy()
task.wait(0.25)
Character.HumanoidRootPart.Anchored = false
LaughAnim:Stop()
end)
SmokeSound:Play()
local Tween = TS:Create(SmokeSound, TweenInfo.new(1.5), {Volume = 0})
Tween:Play()
Tween.Completed:Connect(function()
SmokeSound:Destroy()
end)
Wave:Emit(2)
Smoke:Emit(100)
Pixel:Emit(50)
for _, Item in ipairs(Character.Humanoid:GetAccessories()) do
local Hair = Item.Handle:FindFirstChild("HairAttachment") ~= nil
if Hair then
TextureArray[Item] = {TextureID = Item.Handle.TextureID, Color = Item.Handle.Color, Material = Item.Handle.Material}
Item.Handle.Color = Color3.fromRGB(255, 255, 0)
Item.Handle.TextureID = ''
Item.Handle.Material = Enum.Material.Neon
end
end
elseif Activated then
Activated = false
local Tween = TS:Create(Prop.Handle, TweenInfo.new(0.5), {Transparency = 1})
Tween:Play()
Tween.Completed:Connect(function()
Prop:Destroy()
end)
for _, Item in ipairs(Character.Humanoid:GetAccessories()) do
local Hair = Item.Handle:FindFirstChild("HairAttachment") ~= nil
if Hair then
Item.Handle.Color = TextureArray[Item].Color
Item.Handle.Material = TextureArray[Item].Material
Item.Handle.TextureID = TextureArray[Item].TextureID
TextureArray[Item] = nil
end
end
end
end
end)
|
-----------------------------------------------------------
----------------------- STATIC DATA -----------------------
----------------------------------------------------------- |
local RunService = game:GetService("RunService")
local table = require(script.Parent:WaitForChild("Table"))
local Signal = require(script.Parent:WaitForChild("Signal"))
local FastCast = nil -- Static reference to the FastCast static module.
|
--[=[
Constructs a new Maid which will clean up when the brio dies.
Will error if the Brio is dead.
:::info
Calling this while the brio is already dead will throw a error.
:::
```lua
local brio = Brio.new("a")
local maid = brio:ToMaid()
maid:GiveTask(function()
print("Cleaning up!")
end)
brio:Kill() --> Cleaning up!
```
@return Maid
]=] |
function Brio:ToMaid()
assert(self._values ~= nil, "Brio is dead")
local maid = Maid.new()
maid:GiveTask(self:GetDiedSignal():Connect(function()
maid:DoCleaning()
end))
return maid
end
|
-- Also set this to true if you want the thumbstick to not affect throttle, only triggers when a gamepad is conected |
local onlyTriggersForThrottle = false
local ZERO_VECTOR3 = Vector3.new(0,0,0)
local bindAtPriorityFlagExists, bindAtPriorityFlagEnabled = pcall(function()
return UserSettings():IsUserFeatureEnabled("UserPlayerScriptsBindAtPriority")
end)
local FFlagPlayerScriptsBindAtPriority = bindAtPriorityFlagExists and bindAtPriorityFlagEnabled
|
---Strobe SCRIPT, DO NOT EDIT!--- |
for i,v in pairs(game.workspace.PLights:GetChildren()) do
spawn(function()
while true do
wait(math.random())
v.MLSelling.Dimmer:Invoke(180)
wait(0.001)
v.MLSelling.Dimmer:Invoke(0)
end
end)
end
|
--[[
Manages batch updating of spring objects.
]] |
local RunService = game:GetService("RunService")
local Package = script.Parent.Parent
local PubTypes = require(Package.PubTypes)
local Types = require(Package.Types)
local packType = require(Package.Animation.packType)
local springCoefficients = require(Package.Animation.springCoefficients)
local updateAll = require(Package.Dependencies.updateAll)
local logError = require(Package.Logging.logError)
type Set<T> = {[T]: any}
type Spring = Types.Spring<any>
local SpringScheduler = {}
local WEAK_KEYS_METATABLE = {__mode = "k"}
|
-- If you want to know how to retexture a hat, read this: http://www.roblox.com/Forum/ShowPost.aspx?PostID=10502388 |
debounce = true
function onTouched(hit)
if (hit.Parent:findFirstChild("Humanoid") ~= nil and debounce == true) then
debounce = false
h = Instance.new("Hat")
p = Instance.new("Part")
h.Name = "Handle" -- It doesn't make a difference, but if you want to make your place in Explorer neater, change this to the name of your hat.
p.Parent = h
p.Position = hit.Parent:findFirstChild("Head").Position
p.Name = "Handle"
p.formFactor = 0
p.Size = Vector3.new(2, 2, 2)
p.BottomSurface = 0
p.TopSurface = 0
p.Locked = true
script.Parent.Mesh:clone().Parent = p
h.Parent = hit.Parent
h.AttachmentPos = Vector3.new(0, 0.300000012, 0.219999999) -- Change these to change the positiones of your hat, as I said earlier.
wait(5) debounce = true
end
end
script.Parent.Touched:connect(onTouched)
|
----- Modules |
local CooldownModule = require(RS.Modules.CooldownGui)
|
--[[
Returns the target CFrame for the camera while in Dead mode
]] |
function CameraStateDead.getTargetCFrame(targetPart)
local _, yaw, _ = targetPart.CFrame:toEulerAnglesYXZ()
return CFrame.new(targetPart.CFrame.p) * CFrame.fromEulerAnglesYXZ(0, yaw, 0) * CFrame.new(0, 15, 10) * -- Offset
CFrame.Angles(math.rad(-20), 0, 0) -- Rotation
end
|
--[=[
Retrieves an attribute, and if it is nil, returns the default
instead.
@param instance Instance
@param attributeName string
@param default T?
@return T?
]=] |
function AttributeUtils.getAttribute(instance, attributeName, default)
local value = instance:GetAttribute(attributeName)
if value == nil then
return default
end
return value
end
|
--[=[
@within Plasma
@function checkbox
@tag widgets
@param label string -- The label for the checkbox
@param options {disabled: boolean, checked: boolean}
@return CheckboxWidgetHandle
A checkbox. A checkbox may either be controlled or uncontrolled.
By passing the `checked` field in `options`, you make the checkbox controlled. Controlling the checkbox means that
the checked state is controlled by your code. Otherwise, the controlled state is controlled by the widget itself.
Returns a widget handle, which has the fields:
- `checked`, a function you can call to check if the checkbox is checked
- `clicked`, a function you can call to check if the checkbox was clicked this frame
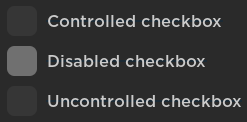
```lua
Plasma.window("Checkboxes", function()
if Plasma.checkbox("Controlled checkbox", {
checked = checked,
}):clicked() then
checked = not checked
end
Plasma.checkbox("Disabled checkbox", {
checked = checked,
disabled = true,
})
Plasma.checkbox("Uncontrolled checkbox")
end)
```
]=] |
local Runtime = require(script.Parent.Parent.Runtime)
local create = require(script.Parent.Parent.create)
return Runtime.widget(function(text, options)
options = options or {}
local checked, setChecked = Runtime.useState(false)
local clicked, setClicked = Runtime.useState(false)
local refs = Runtime.useInstance(function(ref)
local Checkbox = create("Frame", {
[ref] = "checkbox",
BackgroundTransparency = 1,
Name = "Checkbox",
Size = UDim2.new(0, 30, 0, 30),
AutomaticSize = Enum.AutomaticSize.X,
create("TextButton", {
BackgroundColor3 = Color3.fromRGB(54, 54, 54),
BorderSizePixel = 0,
Font = Enum.Font.SourceSansBold,
Size = UDim2.new(0, 30, 0, 30),
TextColor3 = Color3.fromRGB(153, 153, 153),
TextSize = 24,
create("UICorner", {
CornerRadius = UDim.new(0, 8),
}),
Activated = function()
setClicked(true)
setChecked(function(currentlyChecked)
return not currentlyChecked
end)
end,
}),
create("TextLabel", {
BackgroundColor3 = Color3.fromRGB(255, 255, 255),
Font = Enum.Font.GothamMedium,
TextColor3 = Color3.fromRGB(203, 203, 203),
TextSize = 18,
AutomaticSize = Enum.AutomaticSize.X,
RichText = true,
}),
create("UIListLayout", {
FillDirection = Enum.FillDirection.Horizontal,
Padding = UDim.new(0, 10),
SortOrder = Enum.SortOrder.LayoutOrder,
VerticalAlignment = Enum.VerticalAlignment.Center,
}),
})
return Checkbox
end)
local instance = refs.checkbox
instance.TextLabel.Text = text
instance.TextButton.AutoButtonColor = not options.disabled
Runtime.useEffect(function()
local isChecked
if options.checked ~= nil then
isChecked = options.checked
else
isChecked = checked
end
instance.TextButton.Text = isChecked and "✓" or ""
end, options.checked, checked)
Runtime.useEffect(function()
instance.TextButton.BackgroundColor3 = options.disabled and Color3.fromRGB(112, 112, 112)
or Color3.fromRGB(54, 54, 54)
end, options.disabled)
local handle = {
checked = function()
if options.checked or checked then
return true
end
return false
end,
clicked = function()
if clicked then
setClicked(false)
return true
end
return false
end,
}
return handle
end)
|
--DO NOT EDIT BELOW! |
while wait() do
Main.Money.Text = Money.Value
end
|
--[[
Returns the current value of this Spring object.
The object will be registered as a dependency unless `asDependency` is false.
]] |
function class:get(asDependency: boolean?)
if asDependency ~= false then
useDependency(self)
end
return self._currentValue
end
|
--// Commands
--// Highly recommended you disable Intellesense before editing this... |
return function(Vargs)
local server = Vargs.Server;
local service = Vargs.Service;
local Settings = server.Settings
local Functions, Commands, Admin, Anti, Core, HTTP, Logs, Remote, Process, Variables, Deps
local function Init()
Functions = server.Functions;
Admin = server.Admin;
Anti = server.Anti;
Core = server.Core;
HTTP = server.HTTP;
Logs = server.Logs;
Remote = server.Remote;
Process = server.Process;
Variables = server.Variables;
Commands = server.Commands;
Deps = server.Deps;
--// Automatic New Command Caching and Ability to do server.Commands[":ff"]
setmetatable(Commands, {
__index = function(self, ind)
local targInd = Admin.CommandCache[ind:lower()]
if targInd then
return rawget(Commands, targInd)
end
end;
__newindex = function(self, ind, val)
rawset(Commands, ind, val)
if val and type(val) == "table" and val.Commands and val.Prefix then
for i,cmd in next,val.Commands do
Admin.PrefixCache[val.Prefix] = true;
Admin.CommandCache[(val.Prefix..cmd):lower()] = ind;
end
end
end;
})
Logs:AddLog("Script", "Loading Command Modules...")
--// Load command modules
if server.CommandModules then
for i,module in next,server.CommandModules:GetChildren() do
local func = require(module)
local ran,tab = pcall(func, Vargs, getfenv())
if ran and tab and type(tab) == "table" then
for ind,cmd in next,tab do
Commands[ind] = cmd;
end
Logs:AddLog("Script", "Loaded Command Module: ".. module.Name)
elseif not ran then
warn("CMDMODULE ".. module.Name .. " failed to load:")
warn(tostring(tab))
Logs:AddLog("Script", "Loading Command Module Failed: ".. module.Name)
end
end
end
--// Cache commands
Admin.CacheCommands();
Logs:AddLog("Script", "Commands Module Initialized")
end;
server.Commands = {
Init = Init;
};
end
|
-- events |
REMOTES.Kick.OnServerEvent:Connect(function(player, other)
local squad = GetSquad(other)
if squad then
if player.Name == squad.Leader.Value then
LeaveSquad(other)
end
end
end)
REMOTES.SetLeader.OnServerEvent:Connect(function(player, other)
local squad = GetSquad(other)
if squad then
if player.Name == squad.Leader.Value then
squad.Leader.Value = other.Name
end
end
end)
REMOTES.ToggleQueue.OnServerEvent:connect(function(player)
local squad = GetSquad(player)
if squad then
if player.Name == squad.Leader.Value then
if squad.Queue.Value == "Solo" then
squad.Queue.Value = "Squad"
elseif squad.Queue.Value == "Squad" then
if #squad.Players:GetChildren() <= 1 then
squad.Queue.Value = "Solo"
squad.Fill.Value = false
end
end
end
end
end)
REMOTES.ToggleFill.OnServerEvent:connect(function(player)
local squad = GetSquad(player)
if squad then
if player.Name == squad.Leader.Value then
if squad.Queue.Value == "Solo" then
squad.Fill.Value = false
else
squad.Fill.Value = not squad.Fill.Value
end
end
end
end)
REMOTES.SendInvite.OnServerEvent:connect(function(from, to)
local squad = GetSquad(from)
if squad.Leader.Value == from.Name then
if #squad.Players:GetChildren() < MAX_SQUAD_SIZE then
local success = false
pcall(function()
response = REMOTES.RespondToInvite:InvokeClient(to, from.Name)
end)
if response and from:IsDescendantOf(Players) then
local squad = GetSquad(from)
if #squad.Players:GetChildren() < MAX_SQUAD_SIZE then
AddPlayerToSquad(to, squad)
end
end
end
end
end)
REMOTES.Ready.OnServerEvent:connect(function(player, ready)
local squad = GetSquad(player)
if squad then
squad.Players[player.Name].Value = ready
end
end)
REMOTES.LeaveSquad.OnServerEvent:connect(function(player)
LeaveSquad(player)
end)
Players.PlayerAdded:connect(function(player)
local info = player:GetJoinData()
local squadName = info.TeleportData
PLAYER_DATA:WaitForChild(player.Name)
if squadName then
local currentSquad = SQUADS:FindFirstChild(squadName)
if currentSquad then
if #currentSquad.Players:GetChildren() < MAX_SQUAD_SIZE then
AddPlayerToSquad(player, currentSquad)
else
AddPlayerToSquad(player, CreateSquad())
end
else
AddPlayerToSquad(player, CreateSquad(squadName))
end
else
AddPlayerToSquad(player, CreateSquad())
end
end)
Players.PlayerRemoving:connect(function(player)
RemovePlayer(player)
end)
|
--[=[
Accepts an array of Promises and returns a Promise that is resolved as soon as `count` Promises are resolved from the input array. The resolved array values are in the order that the Promises resolved in. When this Promise resolves, all other pending Promises are cancelled if they have no other consumers.
`count` 0 results in an empty array. The resultant array will never have more than `count` elements.
```lua
local promises = {
returnsAPromise("example 1"),
returnsAPromise("example 2"),
returnsAPromise("example 3"),
}
return Promise.some(promises, 2) -- Only resolves with first 2 promises to resolve
```
@param promises {Promise<T>}
@param count number
@return Promise<{T}>
]=] |
function Promise.some(promises, amount)
assert(type(amount) == "number", "Bad argument #2 to Promise.some: must be a number")
return Promise._all(debug.traceback(nil, 2), promises, amount)
end
|
--functions |
function pose1()
local player = game.Players.LocalPlayer
if (player == nil) then return end
char = player.Character
Rshoulder = char.Torso:findFirstChild("Right Shoulder")
Lshoulder = char.Torso:findFirstChild("Left Shoulder")
Rhip = char.Torso:findFirstChild("Right Hip")
Lhip = char.Torso:findFirstChild("Left Hip")
if (Rshoulder ~= nil) then Rshoulder.DesiredAngle = 0 end
if (Lshoulder ~= nil) then Lshoulder.DesiredAngle = 0 end
if (Rhip ~= nil) then Rhip.DesiredAngle = 0 end
if (Lhip ~= nil) then Lhip.DesiredAngle = 0 end
end
function noanimate()
local player = game.Players.LocalPlayer
if (player == nil) then return end
char = player.Character
local Anim = char:findFirstChild("Animate")
if (Anim ~= nil) then
Anim.Parent = player
end
end
function animate()
local player = game.Players.LocalPlayer
if (player == nil) then return end
char = player.Character
local Anim = player:findFirstChild("Animate")
if (Anim ~= nil) then
Anim.Parent = char
wait()
Rshoulder = char.Torso:findFirstChild("Right Shoulder")
Lshoulder = char.Torso:findFirstChild("Left Shoulder")
Rhip = char.Torso:findFirstChild("Right Hip")
Lhip = char.Torso:findFirstChild("Left Hip")
if (Rshoulder ~= nil) then
Rshoulder.Parent = nil
Rshoulder.Parent = char.Torso
end
if (Lshoulder ~= nil) then
Lshoulder.Parent = nil
Lshoulder.Parent = char.Torso
end
if (Rhip ~= nil) then
Rhip.Parent = nil
Rhip.Parent = char.Torso
end
if (Lhip ~= nil) then
Lhip.Parent = nil
Lhip.Parent = char.Torso end
end
end
function FalconPUUUNCH(mouse)
local player = game.Players.LocalPlayer
if (player == nil) then return end
char = player.Character
if (punchload == false) then
Rarm = char:findFirstChild("Right Arm")
Torso = char.Torso
Rshoulder = Torso:findFirstChild("Right Shoulder")
if (Rarm ~= nil) and (Rshoulder ~= nil) then
punchload = true
mouse.Icon = "rbxasset://textures\\GunWaitCursor.png"
noanimate()
pose1()
Rshoulder.MaxVelocity = 1
Rshoulder.DesiredAngle = 0
wait(0.5)
hurtc = hurt:clone()
hurtc.Disabled = false
hurtc.Parent = Rarm
hurtc.Owner.Value = player.Name
Rshoulder.DesiredAngle = 2
wait(0.1)
Rshoulder.MaxVelocity = 0.1
wait(1)
while hurtc.Enabled.Value == true do
wait(0.1)
end
hurtc:remove()
animate()
punchload = false
mouse.Icon = "rbxasset://textures\\GunCursor.png"
end
end
end
|
-- Local Variables |
local IntermissionRunning = false
local EnoughPlayers = false
local GameRunning = false
local Events = ReplicatedStorage.Events
local CaptureFlag = Events.CaptureFlag
local ReturnFlag = Events.ReturnFlag
|
--Glenn's Anti-Exploit System (GAE for short). This code is very ugly, but does job done |
local function SecureSettings(Player, Gun, Module)
if Player then
local PreNewModule = Gun:FindFirstChild("Setting")
if Gun and PreNewModule then
local NewModule = require(PreNewModule)
if (CompareTables(Module, NewModule) == false) then
if KickPlayer then
Player:Kick("You have been kicked and blocked from rejoining this specific server for exploiting gun stats.")
warn(Player.Name.." has been kicked for exploiting gun stats.")
table.insert(_G.TempBannedPlayers, Player)
else
warn(Player.Name.." - Potential Exploiter Bypass! Case 2: Changed Gun Stats From Client")
end
return
end
else
--[[if KickPlayer then
Player:Kick("Gun and Module are not found. Kicked!")
warn("Gun and Module are missing from "..Player.Name.."'s inventory.")
else
warn(Player.Name.." - Potential Exploiter Bypass! Case 1: Missing Gun And Module")
end]]
warn(Player.Name.." - Potential Exploiter Bypass! Case 1: Missing Gun And Module")
return
end
else
warn("Player does not exist.")
return
end
end
function _G.SecureSettings(Player, Gun, Module)
SecureSettings(Player, Gun, Module)
end
VisualizeHitEffect.OnServerEvent:Connect(function(Player, Type, Replicate, Hit, Position, Normal, Material, ...)
local Table = {...}
if Type == "Normal" then
for _, plr in next, Players:GetPlayers() do
if plr ~= Player then
VisualizeHitEffect:FireClient(plr, Type, Replicate, Hit, Position, Normal, Material, Table[1], Table[2], Table[3], nil)
end
end
elseif Type == "Blood" then
for _, plr in next, Players:GetPlayers() do
if plr ~= Player then
VisualizeHitEffect:FireClient(plr, Type, Replicate, Hit, Position, Normal, Material, Table[1], Table[2], nil)
end
end
end
end)
VisualizeBullet.OnServerEvent:Connect(function(Player, Module, Tool, Handle, Directions, FirePointObject, HitEffectData, BloodEffectData, BulletHoleData, ExplosiveData, BulletData, WhizData, ClientData)
for _, plr in next, Players:GetPlayers() do
if plr ~= Player then
VisualizeBullet:FireClient(plr, Module, Tool, Handle, Directions, FirePointObject, HitEffectData, BloodEffectData, BulletHoleData, ExplosiveData, BulletData, WhizData, ClientData)
end
end
end)
VisualizeMuzzle.OnServerEvent:Connect(function(Player, Handle, MuzzleFlashEnabled, MuzzleLightData, MuzzleEffect, Replicate)
for _, plr in next, Players:GetPlayers() do
if plr ~= Player then
VisualizeMuzzle:FireClient(plr, Handle, MuzzleFlashEnabled, MuzzleLightData, MuzzleEffect, Replicate)
end
end
end)
PlayAudio.OnServerEvent:Connect(function(Player, Audio, LowAmmoAudio, Replicate)
for _, plr in next, Players:GetPlayers() do
if plr ~= Player then
PlayAudio:FireClient(plr, Audio, LowAmmoAudio, Replicate)
end
end
end)
ShatterGlass.OnServerEvent:Connect(function(Player, Hit, Pos, Dir)
if Hit then
if Hit.Name == "_glass" then
if Hit.Transparency ~= 1 then
if PhysicEffect then
local Sound = Instance.new("Sound")
Sound.SoundId = "http://roblox.com/asset/?id=2978605361"
Sound.TimePosition = .1
Sound.Volume = 1
Sound.Parent = Hit
Sound:Play()
Sound.Ended:Connect(function()
Sound:Destroy()
end)
GlassShattering:Shatter(Hit, Pos, Dir + Vector3.new(math.random(-25, 25), math.random(-25, 25), math.random(-25, 25)))
--[[local LifeTime = 5
local FadeTime = 1
local SX, SY, SZ = Hit.Size.X, Hit.Size.Y, Hit.Size.Z
for X = 1, 4 do
for Y = 1, 4 do
local Part = Hit:Clone()
local position = Vector3.new(X - 2.1, Y - 2.1, 0) * Vector3.new(SX / 4, SY / 4, SZ)
local currentTransparency = Part.Transparency
Part.Name = "_shatter"
Part.Size = Vector3.new(SX / 4, SY / 4, SZ)
Part.CFrame = Hit.CFrame * (CFrame.new(Part.Size / 8) - Hit.Size / 8 + position)
Part.Velocity = Vector3.new(math.random(-10, 10), math.random(-10, 10), math.random(-10, 10))
Part.Parent = workspace
--Debris:AddItem(Part, 10)
task.delay(LifeTime, function()
if Part.Parent ~= nil then
if LifeTime > 0 then
local t0 = os.clock()
while true do
local Alpha = math.min((os.clock() - t0) / FadeTime, 1)
Part.Transparency = Math.Lerp(currentTransparency, 1, Alpha)
if Alpha == 1 then break end
task.wait()
end
Part:Destroy()
else
Part:Destroy()
end
end
end)
Part.Anchored = false
end
end]]
else
local Sound = Instance.new("Sound")
Sound.SoundId = "http://roblox.com/asset/?id=2978605361"
Sound.TimePosition = .1
Sound.Volume = 1
Sound.Parent = Hit
Sound:Play()
Sound.Ended:Connect(function()
Sound:Destroy()
end)
local Particle = script.Shatter:Clone()
Particle.Color = ColorSequence.new(Hit.Color)
Particle.Transparency = NumberSequence.new{
NumberSequenceKeypoint.new(0, Hit.Transparency), --(time, value)
NumberSequenceKeypoint.new(1, 1)
}
Particle.Parent = Hit
task.delay(0.01, function()
Particle:Emit(10 * math.abs(Hit.Size.magnitude))
Debris:AddItem(Particle, Particle.Lifetime.Max)
end)
Hit.CanCollide = false
Hit.Transparency = 1
end
end
else
error("Hit part's name must be '_glass'.")
end
else
error("Hit part doesn't exist.")
end
end)
local function CalculateDamage(Damage, TravelDistance, ZeroDamageDistance, FullDamageDistance)
local ZeroDamageDistance = ZeroDamageDistance or 10000
local FullDamageDistance = FullDamageDistance or 1000
local DistRange = ZeroDamageDistance - FullDamageDistance
local FallOff = math.clamp(1 - (math.max(0, TravelDistance - FullDamageDistance) / math.max(1, DistRange)), 0, 1)
return math.max(Damage * FallOff, 0)
end
InflictTarget.OnServerInvoke = function(Player, Module, Tool, Tagger, TargetHumanoid, TargetTorso, Damage, Misc, Critical, Hit, GoreData, ExplosiveData)
SecureSettings(Player, Tool, Module) --Second layer
local TrueDamage
if ExplosiveData and ExplosiveData[1] then
local DamageMultiplier = (1 - math.clamp((ExplosiveData[3] / ExplosiveData[2]), 0, 1))
TrueDamage = ExplosiveData[4] and ((Hit and Hit.Name == "Head" and Damage[3]) and Damage[1] * Damage[2] or Damage[1]) * DamageMultiplier or (Hit and Hit.Name == "Head" and Damage[3]) and Damage[1] * Damage[2] or Damage[1]
else
TrueDamage = Damage[5] and CalculateDamage((Hit and Hit.Name == "Head" and Damage[3]) and Damage[1] * Damage[2] or Damage[1], Damage[4], Damage[6], Damage[7]) or (Hit and Hit.Name == "Head" and Damage[3]) and Damage[1] * Damage[2] or Damage[1]
end
--GORE
if TargetHumanoid.Health - TrueDamage <= 0 and not TargetHumanoid.Parent:FindFirstChild("gibbed") then
if Hit then
if Hit.Name == "Head" or Hit.Name == "Torso" or Hit.Name == "Left Arm" or Hit.Name == "Right Arm" or Hit.Name == "Right Leg" or Hit.Name == "Left Leg" or Hit.Name == "UpperTorso" or Hit.Name == "LowerTorso" or Hit.Name == "LeftUpperArm" or Hit.Name == "LeftLowerArm" or Hit.Name == "LeftHand" or Hit.Name == "RightUpperArm" or Hit.Name == "RightLowerArm" or Hit.Name == "RightHand" or Hit.Name == "RightUpperLeg" or Hit.Name == "RightLowerLeg" or Hit.Name == "RightFoot" or Hit.Name == "LeftUpperLeg" or Hit.Name == "LeftLowerLeg" or Hit.Name == "LeftFoot" then
VisualizeGore:FireAllClients(Hit, TargetHumanoid.Parent, GoreData)
end
end
end
if Tagger then
local CanDamage = DamageModule.CanDamage(TargetHumanoid.Parent, Tool.Parent, Damage[8])
if ExplosiveData and ExplosiveData[1] and ExplosiveData[5] then
if TargetHumanoid.Parent.Name == Tagger.Name then
CanDamage = (TargetHumanoid.Parent.Name == Tagger.Name)
if ExplosiveData[7] then
TrueDamage = TargetHumanoid:GetState() ~= Enum.HumanoidStateType.Freefall and TrueDamage or (TrueDamage * (1 - ExplosiveData[6]))
else
TrueDamage = TrueDamage * (1 - ExplosiveData[6])
end
end
end
if TargetHumanoid and TargetHumanoid.Health ~= 0 and TargetTorso and CanDamage then
while TargetHumanoid:FindFirstChild("creator") do
TargetHumanoid.creator:Destroy()
end
local creator = Instance.new("ObjectValue",TargetHumanoid)
creator.Name = "creator"
creator.Value = Tagger
Debris:AddItem(creator, 5)
if Critical[1] then
local CriticalChanceRandom = Random.new():NextInteger(0, 100)
if CriticalChanceRandom <= Critical[2] then
TargetHumanoid:TakeDamage(math.abs(TrueDamage * Critical[3]))
else
TargetHumanoid:TakeDamage(math.abs(TrueDamage))
end
else
TargetHumanoid:TakeDamage(math.abs(TrueDamage))
end
if Misc[1] > 0 then --knockback
if not (ExplosiveData and ExplosiveData[1] and ExplosiveData[5]) then
local Shover = Tagger.Character.HumanoidRootPart or Tagger.Character.Head
local Duration = 0.1
local Speed = Misc[1] / Duration
local Velocity = (TargetTorso.Position - Shover.Position).Unit * Speed
local ShoveForce = Instance.new("BodyVelocity")
ShoveForce.MaxForce = Vector3.new(1e9, 1e9, 1e9)
ShoveForce.Velocity = Velocity
ShoveForce.Parent = TargetTorso
Debris:AddItem(ShoveForce, Duration)
end
end
if Misc[2] > 0 and Tagger.Character.Humanoid and Tagger.Character.Humanoid.Health ~= 0 then --lifesteal
if ExplosiveData and ExplosiveData[1] and ExplosiveData[5] then
if not TargetHumanoid.Parent.Name == Tagger.Name then
Tagger.Character.Humanoid.Health = Tagger.Character.Humanoid.Health + (TrueDamage * Misc[2])
end
else
Tagger.Character.Humanoid.Health = Tagger.Character.Humanoid.Health + (TrueDamage * Misc[2])
end
end
if Misc[3] then --affliction
local roll = math.random(1, 100)
if roll <= Misc[5] then
if not TargetHumanoid.Parent:FindFirstChild(Misc[4]) then
local Debuff = Tool:FindFirstChild("GunScript_Server"):FindFirstChild(Misc[4]):Clone()
Debuff.creator.Value = creator.Value
Debuff.Parent = TargetHumanoid.Parent
Debuff.Disabled = false
end
end
end
end
else
warn("Unable to register damage because player is no longer existing here")
end
end
Players.PlayerAdded:Connect(function(player)
for i, v in pairs(_G.TempBannedPlayers) do
if v == player.Name then
player:Kick("You cannot rejoin a server where you were kicked from.")
warn(player.Name.." tried to rejoin a server where he/she was kicked from.")
break
end
end
end)
|
-- ================================================================================
-- PUBLIC FUNCTIONS
-- ================================================================================ |
function GuiController:UpdateSpeed()
end -- GuiController:UpdateSpeed()
function GuiController:Start(s)
speeder = s
primary = speeder.PrimaryPart
engine = speeder.engine
BoostSetup()
end -- GuiController:Start()
function GuiController:Stop()
end -- GuiController:Stop() |
--METHOD 1 PARAMS |
local CALMDOWN = 0.5
local CALMDOWN_RENDER = nil |
-------------------------------------------------------------------------------- |
local function SetWaterState(ReadyToUse: boolean)
for _, WaterPart in Fountain.Water:GetChildren() do
if WaterPart:IsA("BasePart") then
if not WaterColor[WaterPart] then
WaterColor[WaterPart] = WaterPart.Color
end
Tween:Play(WaterPart, {1, "Circular"}, {Color = ReadyToUse and WaterColor[WaterPart] or Color3.fromRGB(118, 118, 118)})
end
end
end
Fountain.Healed.OnClientEvent:Connect(function(ServerTime: number)
SetWaterState(false)
Tween:Play(BillboardGui.Header, {1, "Circular"}, {TextTransparency = 0.7})
BillboardGui.Cooldown.Visible = true
while true do
local Difference = workspace:GetServerTimeNow() - ServerTime
local ReadyToUse = Difference > Timer
if ReadyToUse then
break
end
BillboardGui.Cooldown.Text = "-".. math.ceil(Timer-Difference) .."-"
task.wait(0.2)
end
SetWaterState(true)
Tween:Play(BillboardGui.Header, {1, "Circular"}, {TextTransparency = 0})
BillboardGui.Cooldown.Visible = false
end)
|
--[[
A limited, simple implementation of a Signal.
Handlers are fired in order, and (dis)connections are properly handled when
executing an event.
]] |
local function immutableAppend(list, ...)
local new = {}
local len = #list
for key = 1, len do
new[key] = list[key]
end
for i = 1, select("#", ...) do
new[len + i] = select(i, ...)
end
return new
end
local function immutableRemoveValue(list, removeValue)
local new = {}
for i = 1, #list do
if list[i] ~= removeValue then
table.insert(new, list[i])
end
end
return new
end
local Signal = {}
Signal.__index = Signal
function Signal.new()
local self = {
_listeners = {}
}
setmetatable(self, Signal)
return self
end
function Signal:connect(callback)
local listener = {
callback = callback,
disconnected = false,
}
self._listeners = immutableAppend(self._listeners, listener)
local function disconnect()
listener.disconnected = true
self._listeners = immutableRemoveValue(self._listeners, listener)
end
return {
disconnect = disconnect
}
end
function Signal:fire(...)
for _, listener in ipairs(self._listeners) do
if not listener.disconnected then
listener.callback(...)
end
end
end
return Signal
|
-- 2xy0x |
local module = {}
module.LastStandingPos = nil;
module.DontUpdateYet = false;
module.DamageMultiplication = 0.065
module.MinimumFallDamageStuds = 90
return module
|
--[[
Updates the value stored in this State object.
If `force` is enabled, this will skip equality checks and always update the
state object and any dependents - use this with care as this can lead to
unnecessary updates.
]] |
function class:set(newValue: any, force: boolean?)
-- if the value hasn't changed, no need to perform extra work here
if self._value == newValue and not force then
return
end
self._value = newValue
-- update any derived state objects if necessary
updateAll(self)
end
local function Value<T>(initialValue: T): Types.State<T>
local self = setmetatable({
type = "State",
kind = "State",
-- if we held strong references to the dependents, then they wouldn't be
-- able to get garbage collected when they fall out of scope
dependentSet = setmetatable({}, WEAK_KEYS_METATABLE),
_value = initialValue
}, CLASS_METATABLE)
initDependency(self)
return self
end
return Value
|
-- Get references to the DockShelf and its children |
local dockShelf = script.Parent.Parent.Parent.Parent.Parent.DockShelf
local dockbtn = dockShelf.JCookie
local aFinderButton = dockShelf.AFinder
local Minimalise = script.Parent
local window = script.Parent.Parent.Parent
|
--[Lokalizacje]
--[Góra] |
g1 = script.Parent.Parent.gura1
legansio = false
g2 = script.Parent.Parent.gura2
legansio = false
g3 = script.Parent.Parent.gura3
legansio = false
g4 = script.Parent.Parent.gura4
legansio = false
g5 = script.Parent.Parent.gura5
legansio = false
g6 = script.Parent.Parent.gura6
legansio = false
g7 = script.Parent.Parent.gura7
legansio = false
g8 = script.Parent.Parent.gura8
legansio = false
g9 = script.Parent.Parent.gura9
legansio = false
g10 = script.Parent.Parent.gura10
legansio = false
g11 = script.Parent.Parent.gura11
legansio = false
g12 = script.Parent.Parent.gura12
legansio = false
g13 = script.Parent.Parent.gura13
legansio = false
g14 = script.Parent.Parent.gura14
legansio = false
s1 = script.Parent.Parent.s1
legansio = false
q1 = script.Parent.Parent.q1
legansio = false |
--[[
Package link auto-generated by Rotriever
]] |
local PackageIndex = script.Parent.Parent.Parent._Index
local Package = require(PackageIndex["JestMatcherUtils"]["JestMatcherUtils"])
export type MatcherHintOptions = Package.MatcherHintOptions
export type DiffOptions = Package.DiffOptions
return Package
|
-- Tags -- |
local pantsId = script.Parent.Parent.ClickPart.Pants.PantsTemplate
local shirtId = script.Parent.Parent.ClickPart.Shirt.ShirtTemplate
local cPart = script.Parent
local cDetector = script.Parent.ClickDetector
|
-- Don't change the wait time. Sorry, it breaks the script... | |
-- Implements equivalent functionality to JavaScript's `array.indexOf`,
-- implementing the interface and behaviors defined at:
-- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/indexOf
--
-- This implementation is loosely based on the one described in the polyfill
-- source in the above link |
return function<T>(array: Array<T>, searchElement: any, fromIndex: number?): number
local fromIndex_ = fromIndex or 1
local length = #array
-- In the JS impl, a negative fromIndex means we should use length - index;
-- with Lua, of course, this means that 0 is still valid, but refers to the
-- end of the array the way that '-1' would in JS
if fromIndex_ < 1 then
fromIndex_ = math.max(length - math.abs(fromIndex_), 1)
end
for i = fromIndex_, length do
if array[i] == searchElement then
return i
end
end
return -1
end
|
-- goro7 |
local plr = game.Players.LocalPlayer
function update()
-- Calculate scale
local scale = (plr.PlayerGui.MainGui.AbsoluteSize.Y - 30) / script.Parent.Parent.Size.Y.Offset
if scale > 1.5 then
scale = 1.5
end
if scale > 0 then
script.Parent.Scale = scale
end
end
plr.PlayerGui:WaitForChild("MainGui"):GetPropertyChangedSignal("AbsoluteSize"):connect(update)
update()
|
--Enum.SavedQualitySetting.Automatic |
repeat wait() until game.Players.LocalPlayer and game.Players.LocalPlayer.Character and game.Players.LocalPlayer.Character:FindFirstChild("Head")
wait(1)
local Camera=game.Workspace.CurrentCamera
if Camera:FindFirstChild("MotionBlur") then
Camera.MotionBlur:Destroy()
end
local blur=Instance.new("BlurEffect")
blur.Parent=Camera
blur.Size=0
blur.Name="MotionBlur"
local move_anim_speed = 3
local last_p = Vector3.new()
local move_amm = 0
local aim_settings = {
aim_amp = 0.5,
aim_max_change = 4,
aim_retract = 15,
aim_max_deg = 20,
}
local last_va = 0
local last_va2 = 0
local view_velocity = {0, 0}
local last_time = tick()
game:GetService("RunService"):UnbindFromRenderStep("MotionBlur")
wait(1/30)
game:GetService("RunService"):BindToRenderStep("MotionBlur", Enum.RenderPriority.Camera.Value-1, function()
local delta = tick() - last_time
last_time = tick()
local p_distance = ((Camera.CoordinateFrame.lookVector)).magnitude
if p_distance == 0 then p_distance = 0.0001 end
local p_height = Camera.CoordinateFrame.lookVector.y
local view_angle
if p_height ~= 0 then
view_angle = math.deg(math.asin(math.abs(p_height) / p_distance)) * (math.abs(p_height) / p_height)
else
view_angle = 0
end
local cam_cf = Camera.CoordinateFrame
local looking_at = cam_cf * CFrame.new(0, 0, -100)
local view_angle2 = math.deg(math.atan2(cam_cf.p.x - looking_at.p.x, cam_cf.p.z - looking_at.p.z)) + 180
local v_delta1, v_delta2
local dir1, dir2 = 0, 0
v_delta1 = math.abs(view_angle - last_va)
if v_delta1 ~= 0 then
dir1 = (view_angle - last_va) / v_delta1
end
local va_check = {math.abs(view_angle2 - last_va2), 360 - math.abs(view_angle2 - last_va2)}
if view_angle2 == last_va2 then
dir2 = 0
v_delta2 = 0
elseif va_check[1] < va_check[2] then
v_delta2 = va_check[1]
dir2 = (view_angle2 - last_va2) / va_check[1]
else
v_delta2 = va_check[2]
if last_va2 > view_angle2 then
dir2 = 1
else
dir2 = -1
end
end
last_va = view_angle
last_va2 = view_angle2
view_velocity[1] = view_velocity[1] / (1 + (delta * aim_settings.aim_retract))
view_velocity[2] = view_velocity[2] / (1 + (delta * aim_settings.aim_retract))
local calc1 = v_delta1 * dir1 * aim_settings.aim_amp
if calc1 ~= 0 then
view_velocity[1] = view_velocity[1] + (math.abs(calc1)) * (calc1 / math.abs(calc1))
end
local calc2 = v_delta2 * dir2 * aim_settings.aim_amp
if calc2 ~= 0 then
view_velocity[2] = view_velocity[2] + (math.abs(calc2)) * (calc2 / math.abs(calc2))
end
if view_velocity[1] ~= 0 then
view_velocity[1] = math.abs(view_velocity[1]) * (math.abs(view_velocity[1]) / view_velocity[1])
end
if view_velocity[2] ~= 0 then
view_velocity[2] = math.abs(view_velocity[2]) * (math.abs(view_velocity[2]) / view_velocity[2])
end
local one=math.abs(math.rad(view_velocity[1]))
local two=math.abs(math.rad(view_velocity[2]))
local equation=math.max(one,two)*10
blur.Size=equation
if game.Players.LocalPlayer.Character then
if game.Players.LocalPlayer.Character:FindFirstChild("Torso") then
if game.Players.LocalPlayer.Character.Torso.Position.Y <= -2000 then
game.Players.LocalPlayer.Character.Humanoid.Health = 0
end
end
end
end)
|
---Size |
local FSX = Frame.Size.X.Scale
local FSY = Frame.Size.Y.Scale
Player.DataFolder.Points.Changed:Connect(function()
StatTrack.Text = Points.Value
local function GoBack()
Frame:TweenSize(
UDim2.new(FSX,0,FSY,0),
Enum.EasingDirection.Out,
Enum.EasingStyle.Back,
0.125,
true
)
end
Frame:TweenSize(
UDim2.new(FSX + 0.019 ,0,FSY + 0.019,0),
Enum.EasingDirection.Out,
Enum.EasingStyle.Back,
0.125,
true,
GoBack
)
end)
|
--------LEFT DOOR 7-------- |
game.Workspace.doorleft.l71.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l72.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l73.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l61.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l62.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l63.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l52.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l53.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l51.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l43.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l42.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l41.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l33.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l32.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l31.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l23.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l22.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l13.BrickColor = BrickColor.new(135)
game.Workspace.doorleft.l11.BrickColor = BrickColor.new(102)
game.Workspace.doorleft.l12.BrickColor = BrickColor.new(102)
game.Workspace.doorleft.l21.BrickColor = BrickColor.new(102)
|
--Serivice |
game:GetService('StarterGui'):SetCoreGuiEnabled(Enum.CoreGuiType.Backpack, false) |
--Info.WhiteList = {"Master3395","Itscapcake","thecookieboiyt"} |
Info.WhiteList = {
["Master3395"] = true,
[""] = true,
}
function Info:Convert(object)
if object.ClassName == "ImageLabel" then
object.ImageTransparency = 1
end
for _,obj in pairs(object:GetDescendants()) do
if obj.ClassName == "ImageLabel" or obj.ClassName == "ImageButton" then
obj.ImageTransparency = 1
elseif obj.ClassName == "TextLabel" then
obj.TextTransparency = 1
obj.TextStrokeTransparency = 1
elseif obj.ClassName == "TextBox" then
obj.TextTransparency = 1
obj.TextStrokeTransparency = 1
obj.BackgroundTransparency = 1
end
end
object.Visible = false
end
function Info:Menu(object,visible,length)
local TweenService = game:GetService("TweenService")
local tweenInfo = TweenInfo.new(length,Enum.EasingStyle.Linear)
if object.ClassName == "ImageLabel" then
if visible == true then
local info = {}
info.ImageTransparency = 0
local tween = TweenService:Create(object,tweenInfo,info)
tween:Play()
else
local info = {}
info.ImageTransparency = 1
local tween = TweenService:Create(object,tweenInfo,info)
tween:Play()
end
end
for _,obj in pairs(object:GetDescendants()) do
if obj.ClassName == "ImageLabel" or obj.ClassName == "ImageButton" then
if visible == true then
local info = {}
info.ImageTransparency = 0
local tween = TweenService:Create(obj,tweenInfo,info)
tween:Play()
else
local info = {}
info.ImageTransparency = 1
local tween = TweenService:Create(obj,tweenInfo,info)
tween:Play()
end
elseif obj.ClassName == "TextLabel" then
if visible == true then
local info = {}
info.TextTransparency = 0
info.TextStrokeTransparency = 0
local tween = TweenService:Create(obj,tweenInfo,info)
tween:Play()
else
local info = {}
info.TextTransparency = 1
info.TextStrokeTransparency = 1
local tween = TweenService:Create(obj,tweenInfo,info)
tween:Play()
end
elseif obj:IsA("TextBox") then
if visible == true then
local info = {}
info.TextTransparency = 0
info.TextStrokeTransparency = 0
info.BackgroundTransparency = 0
local tween = TweenService:Create(obj,tweenInfo,info)
tween:Play()
else
local info = {}
info.TextTransparency = 1
info.TextStrokeTransparency = 1
info.BackgroundTransparency = 1
local tween = TweenService:Create(obj,tweenInfo,info)
tween:Play()
end
end
end
if visible == false then
object.Visible = false
else
object.Visible = true
end
end
function Info:IsDev(player)
if Info.WhiteList[player.Name] then
return true
else
return false
end
end
Info:Convert(frame)
Info:Convert(kickUI)
Info:Convert(banUI)
Info:Convert(resetUI)
Info:Convert(gamepassUI)
local player = game.Players.LocalPlayer
local length = 0.5
button.MouseButton1Click:Connect(function()
if Opened == false then
Opened = true
Info:Menu(frame,true,length)
Info:Menu(resetUI,false,length)
Info:Menu(kickUI,false,length)
Info:Menu(banUI,false,length)
Info:Menu(gamepassUI,false,length)
else
Opened = false
Info:Menu(frame,false,length)
Info:Menu(resetUI,false,length)
Info:Menu(kickUI,false,length)
Info:Menu(banUI,false,length)
Info:Menu(gamepassUI,false,length)
end
end)
frame.Reset.MouseButton1Click:Connect(function()
Info:Menu(resetUI,true,length)
Info:Menu(frame,false,length)
end)
frame.Kick.MouseButton1Click:Connect(function()
Info:Menu(kickUI,true,length)
Info:Menu(frame,false,length)
end)
frame.Ban.MouseButton1Click:Connect(function()
Info:Menu(banUI,true,length)
Info:Menu(frame,false,length)
end)
frame.Gamepass.MouseButton1Click:Connect(function()
Info:Menu(gamepassUI,true,length)
Info:Menu(frame,false,length)
end)
banUI.Ok.MouseButton1Click:Connect(function()
local plrN = banUI.PlayerName.Text
game.ReplicatedStorage.Special:InvokeServer("Ban",plrN)
Info:Menu(banUI,false,length)
Info:Menu(frame,false,length)
Opened = false
end)
kickUI.Ok.MouseButton1Click:Connect(function()
local plrN = kickUI.PlayerName.Text
game.ReplicatedStorage.Special:InvokeServer("Kick",plrN)
Info:Menu(kickUI,false,length)
Info:Menu(frame,false,length)
Opened = false
end)
resetUI.Ok.MouseButton1Click:Connect(function()
local plrN = resetUI.PlayerName.Text
game.ReplicatedStorage.Special:InvokeServer("Reset",plrN)
Info:Menu(resetUI,false,length)
Info:Menu(frame,false,length)
Opened = false
end)
gamepassUI.Ok.MouseButton1Click:Connect(function()
local plrN = gamepassUI.PlayerName.Text
local id = gamepassUI.Gamepass.Text
game.ReplicatedStorage.Special:InvokeServer("Gamepass",plrN,id)
Info:Menu(gamepassUI,false,length)
Info:Menu(frame,false,length)
Opened = false
end)
if Info:IsDev(player) then
gui.Enabled = true
else
gui.Enabled = false
end
while wait() do
if gui.Enabled == true and Info:IsDev(player) == false then
player:Kick("No open this menu.")
end
end
|
-- loop |
local count = 0
local startTick = tick()
while true do
local timeElapsed = tick() - startTick
-- if the game has loaded and the minimum time has elapsed then break the loop
if game:IsLoaded() and timeElapsed > MIN_TIME and game.Workspace.Finish.Value==1 then
break
end
-- animate the text
textLabel.Text = "Loading " .. string.rep(".",count)
count = (count + 1) % 4
wait(.3)
end
game.Workspace.Finish.Value=2
if not game:IsLoaded() then
game.Loaded:Wait()
end |
--nextsound=0 |
nextjump=0
chasing=false
variance=4
damage=11
attackrange=4.5
sightrange=60
runspeed=18
wonderspeed=8
healthregen=false
colors={"Sand red","Dusty Rose","Medium blue","Sand blue","Lavender","Earth green","Brown","Medium stone grey","Brick yellow"}
function raycast(spos,vec,currentdist)
local hit2,pos2=game.Workspace:FindPartOnRay(Ray.new(spos+(vec*.01),vec*currentdist),sp)
if hit2 and pos2 then
if hit2.Transparency>=.8 or hit2.Name=="Handle" or string.sub(hit2.Name,1,6)=="Effect" then
local currentdist=currentdist-(pos2-spos).magnitude
return raycast(pos2,vec,currentdist)
end
end
return hit2,pos2
end
function waitForChild(parent,childName)
local child=parent:findFirstChild(childName)
if child then return child end
while true do
child=parent.ChildAdded:wait()
if child.Name==childName then return child end
end
end
|
-- Factor of torque applied to change the wheel direction
-- Larger number generally means faster braking |
local BRAKING_TORQUE = 16000
|
-- Initialize mesh tool |
local MeshTool = require(CoreTools:WaitForChild 'Mesh')
Core.AssignHotkey('H', Core.Support.Call(Core.EquipTool, MeshTool));
Core.AddToolButton(Core.Assets.MeshIcon, 'H', MeshTool)
|
-- Runs when someone touches our pet |
function touch(part)
-- IF player AND not dead AND has no master
if part.Parent:FindFirstChild('Humanoid') and part.Parent:FindFirstChild('Humanoid').health > 0 and not master then
-- Assign the master
master = part.Parent.Head
--Create a new spring
spring = Instance.new("SpringConstraint", pet)
spring.Attachment0 = pet.SpringAttach
spring.Attachment1 = master.HatAttachment
spring.FreeLength = maxDistance
spring.Stiffness = 1000
spring.Damping = 2
-- Makes pet turn with player
orient = Instance.new("AlignOrientation", pet)
orient.Attachment0 = pet.OrientAttach
orient.Attachment1 = master.HatAttachment
orient.RigidityEnabled = true
-- Reset pet apon death
function death()
spring:Destroy()
orient:Destroy()
pet.CFrame = startpos
master = nil
end
master.Parent.Humanoid.Died:Connect(death)
end
end
pet.Touched:Connect(touch)
|
-- ربط الدالة بحدث الاصطدام |
plank.Touched:Connect(onTouched)
|
-- Make sure to spell it 100% correct. |
local permissions = {
cmds = 'Players',
help = 'Players',
m = 'Moderators',
kick = 'Moderators',
}
local defaultLevel = 'Creators' -- The permission level that will be set for commands not configured above.
|
-- Note that VehicleController does not derive from BaseCharacterController, it is a special case |
local VehicleController = {}
VehicleController.__index = VehicleController
function VehicleController.new(CONTROL_ACTION_PRIORITY)
local self = setmetatable({}, VehicleController)
self.CONTROL_ACTION_PRIORITY = CONTROL_ACTION_PRIORITY
self.enabled = false
self.vehicleSeat = nil
self.throttle = 0
self.steer = 0
self.acceleration = 0
self.decceleration = 0
self.turningRight = 0
self.turningLeft = 0
self.vehicleMoveVector = ZERO_VECTOR3
return self
end
function VehicleController:BindContextActions()
if useTriggersForThrottle then
ContextActionService:BindActionAtPriority("throttleAccel", (function(actionName, inputState, inputObject)
self:OnThrottleAccel(actionName, inputState, inputObject)
return Enum.ContextActionResult.Pass
end), false, self.CONTROL_ACTION_PRIORITY, Enum.KeyCode.ButtonR2)
ContextActionService:BindActionAtPriority("throttleDeccel", (function(actionName, inputState, inputObject)
self:OnThrottleDeccel(actionName, inputState, inputObject)
return Enum.ContextActionResult.Pass
end), false, self.CONTROL_ACTION_PRIORITY, Enum.KeyCode.ButtonL2)
end
ContextActionService:BindActionAtPriority("arrowSteerRight", (function(actionName, inputState, inputObject)
self:OnSteerRight(actionName, inputState, inputObject)
return Enum.ContextActionResult.Pass
end), false, self.CONTROL_ACTION_PRIORITY, Enum.KeyCode.Right)
ContextActionService:BindActionAtPriority("arrowSteerLeft", (function(actionName, inputState, inputObject)
self:OnSteerLeft(actionName, inputState, inputObject)
return Enum.ContextActionResult.Pass
end), false, self.CONTROL_ACTION_PRIORITY, Enum.KeyCode.Left)
end
function VehicleController:Enable(enable, vehicleSeat)
if enable == self.enabled and vehicleSeat == self.vehicleSeat then
return
end
self.vehicleMoveVector = ZERO_VECTOR3
if enable then
if vehicleSeat then
self.vehicleSeat = vehicleSeat
if FFlagPlayerScriptsBindAtPriority then
self:BindContextActions()
else
if useTriggersForThrottle then
ContextActionService:BindAction("throttleAccel", (function(actionName, inputState, inputObject)
self:OnThrottleAccel(actionName, inputState, inputObject)
return Enum.ContextActionResult.Pass
end), false, Enum.KeyCode.ButtonR2)
ContextActionService:BindAction("throttleDeccel", (function(actionName, inputState, inputObject)
self:OnThrottleDeccel(actionName, inputState, inputObject)
return Enum.ContextActionResult.Pass
end), false, Enum.KeyCode.ButtonL2)
end
ContextActionService:BindAction("arrowSteerRight", (function(actionName, inputState, inputObject)
self:OnSteerRight(actionName, inputState, inputObject)
return Enum.ContextActionResult.Pass
end), false, Enum.KeyCode.Right)
ContextActionService:BindAction("arrowSteerLeft", (function(actionName, inputState, inputObject)
self:OnSteerLeft(actionName, inputState, inputObject)
return Enum.ContextActionResult.Pass
end), false, Enum.KeyCode.Left)
end
end
else
if useTriggersForThrottle then
ContextActionService:UnbindAction("throttleAccel")
ContextActionService:UnbindAction("throttleDeccel")
end
ContextActionService:UnbindAction("arrowSteerRight")
ContextActionService:UnbindAction("arrowSteerLeft")
self.vehicleSeat = nil
end
end
function VehicleController:OnThrottleAccel(actionName, inputState, inputObject)
self.acceleration = (inputState ~= Enum.UserInputState.End) and -1 or 0
self.throttle = self.acceleration + self.decceleration
end
function VehicleController:OnThrottleDeccel(actionName, inputState, inputObject)
self.decceleration = (inputState ~= Enum.UserInputState.End) and 1 or 0
self.throttle = self.acceleration + self.decceleration
end
function VehicleController:OnSteerRight(actionName, inputState, inputObject)
self.turningRight = (inputState ~= Enum.UserInputState.End) and 1 or 0
self.steer = self.turningRight + self.turningLeft
end
function VehicleController:OnSteerLeft(actionName, inputState, inputObject)
self.turningLeft = (inputState ~= Enum.UserInputState.End) and -1 or 0
self.steer = self.turningRight + self.turningLeft
end
|
--Weld stuff here |
MakeWeld(car.Misc.Tach.M,car.DriveSeat,"Motor").Name="M"
ModelWeld(misc.Tach.Parts,misc.Tach.M)
MakeWeld(car.Misc.Speedo.N,car.DriveSeat,"Motor").Name="N"
ModelWeld(misc.Speedo.Parts,misc.Speedo.N)
MakeWeld(car.Misc.Wheel.W,car.DriveSeat,"Motor").Name="W"
ModelWeld(car.Misc.Wheel.Parts,car.Misc.Wheel.W)
car.DriveSeat.ChildAdded:connect(function(child)
if child.Name=="SeatWeld" and child:IsA("Weld") and game.Players:GetPlayerFromCharacter(child.Part1.Parent)~=nil then
child.C0=CFrame.new(0,-.5,0)*CFrame.fromEulerAnglesXYZ(-(math.pi/2),0,0)*CFrame.Angles(math.rad(13),0,0)
end
end)
|
-- LocalScript |
local numValue = Player.Eventos.EasterEvent -- substitua "NumValue" pelo nome da sua NumberValue
local textLabel = script.Parent -- substitua "TextLabel" pelo nome do seu TextLabel
|
--// Aim|Zoom|Sensitivity Customization |
ZoomSpeed = 0.20; -- The lower the number the slower and smoother the tween
AimZoom = 15; -- Default zoom
AimSpeed = 0.24;
UnaimSpeed = 0.20;
CycleAimZoom = 30; -- Cycled zoom
MouseSensitivity = 0.25; -- Number between 0.1 and 1
SensitivityIncrement = 0.05; -- No touchy
|
--Simple function to make the door appear and disappear |
local clickDetector = script.Parent.ClickDetector
function onMouseClick()
if script.Parent.CanCollide == false then
print "Close door"
script.Parent.CanCollide = true
script.Parent.Transparency = 0
else
print "Open door"
script.Parent.CanCollide = false
script.Parent.Transparency = 1
end
end
clickDetector.MouseClick:connect(onMouseClick)
|
--[=[
@within TableUtil
@function Copy
@param tbl table -- Table to copy
@param deep boolean? -- Whether or not to perform a deep copy
@return table
Creates a copy of the given table. By default, a shallow copy is
performed. For deep copies, a second boolean argument must be
passed to the function.
:::caution No cyclical references
Deep copies are _not_ protected against cyclical references. Passing
a table with cyclical references _and_ the `deep` parameter set to
`true` will result in a stack-overflow.
]=] |
local function Copy(t: Table, deep: boolean?): Table
if deep then
local function DeepCopy(tbl)
local tCopy = table.create(#tbl)
for k,v in pairs(tbl) do
if type(v) == "table" then
tCopy[k] = DeepCopy(v)
else
tCopy[k] = v
end
end
return tCopy
end
return DeepCopy(t)
else
if #t > 0 then
return table.move(t, 1, #t, 1, table.create(#t))
else
local tCopy = {}
for k,v in pairs(t) do
tCopy[k] = v
end
return tCopy
end
end
end
|
-- Decompiled with the Synapse X Luau decompiler. |
local v1 = {};
v1.__index = v1;
v1.CameraShakeState = {
FadingIn = 0,
FadingOut = 1,
Sustained = 2,
Inactive = 3
};
local l__Vector3_new__1 = Vector3.new;
function v1.new(p1, p2, p3, p4)
if p3 == nil then
p3 = 0;
end;
if p4 == nil then
p4 = 0;
end;
assert(type(p1) == "number", "Magnitude must be a number");
assert(type(p2) == "number", "Roughness must be a number");
assert(type(p3) == "number", "FadeInTime must be a number");
assert(type(p4) == "number", "FadeOutTime must be a number");
local v2 = {
Magnitude = p1,
Roughness = p2,
PositionInfluence = l__Vector3_new__1(),
RotationInfluence = l__Vector3_new__1(),
DeleteOnInactive = true,
roughMod = 1,
magnMod = 1,
fadeOutDuration = p4,
fadeInDuration = p3,
sustain = p3 > 0
};
if p3 > 0 then
local v3 = 0;
else
v3 = 1;
end;
v2.currentFadeTime = v3;
v2.tick = Random.new():NextNumber(-100, 100);
v2._camShakeInstance = true;
return setmetatable(v2, v1);
end;
local l__math_noise__2 = math.noise;
function v1.UpdateShake(p5, p6)
local l__tick__4 = p5.tick;
local v5 = p5.currentFadeTime;
if p5.fadeInDuration > 0 and p5.sustain then
if v5 < 1 then
v5 = v5 + p6 / p5.fadeInDuration;
elseif p5.fadeOutDuration > 0 then
p5.sustain = false;
end;
end;
if not p5.sustain then
v5 = v5 - p6 / p5.fadeOutDuration;
end;
if p5.sustain then
p5.tick = l__tick__4 + p6 * p5.Roughness * p5.roughMod;
else
p5.tick = l__tick__4 + p6 * p5.Roughness * p5.roughMod * v5;
end;
p5.currentFadeTime = v5;
return l__Vector3_new__1(l__math_noise__2(l__tick__4, 0) * 0.5, l__math_noise__2(0, l__tick__4) * 0.5, l__math_noise__2(l__tick__4, l__tick__4) * 0.5) * p5.Magnitude * p5.magnMod * v5;
end;
function v1.StartFadeOut(p7, p8)
if p8 == 0 then
p7.currentFadeTime = 0;
end;
p7.fadeOutDuration = p8;
p7.fadeInDuration = 0;
p7.sustain = false;
end;
function v1.StartFadeIn(p9, p10)
if p10 == 0 then
p9.currentFadeTime = 1;
end;
p9.fadeInDuration = p10 or p9.fadeInDuration;
p9.fadeOutDuration = 0;
p9.sustain = true;
end;
function v1.GetScaleRoughness(p11)
return p11.roughMod;
end;
function v1.SetScaleRoughness(p12, p13)
p12.roughMod = p13;
end;
function v1.GetScaleMagnitude(p14)
return p14.magnMod;
end;
function v1.SetScaleMagnitude(p15, p16)
p15.magnMod = p16;
end;
function v1.GetNormalizedFadeTime(p17)
return p17.currentFadeTime;
end;
function v1.IsShaking(p18)
local v6 = true;
if not (p18.currentFadeTime > 0) then
v6 = p18.sustain;
end;
return v6;
end;
function v1.IsFadingOut(p19)
return not p19.sustain and p19.currentFadeTime > 0;
end;
function v1.IsFadingIn(p20)
local v7 = false;
if p20.currentFadeTime < 1 then
v7 = p20.sustain and p20.fadeInDuration > 0;
end;
return v7;
end;
function v1.GetState(p21)
if p21:IsFadingIn() then
return v1.CameraShakeState.FadingIn;
end;
if p21:IsFadingOut() then
return v1.CameraShakeState.FadingOut;
end;
if p21:IsShaking() then
return v1.CameraShakeState.Sustained;
end;
return v1.CameraShakeState.Inactive;
end;
return v1;
|
--// WIP |
local function LoadPackage(package, folder, runNow)
--// runNow - Run immediately after unpacking (default behavior is to just unpack (((only needed if loading after startup))))
--// runNow currently not used (limitations) so all packages must be present at server startup
local function unpackFolder(curFolder, unpackInto)
if unpackInto then
for _, obj in ipairs(curFolder:GetChildren()) do
local clone = obj:Clone()
if obj:IsA("Folder") then
local realFolder = unpackInto:FindFirstChild(obj.Name)
if not realFolder then
clone.Parent = unpackInto
else
unpackFolder(obj, realFolder)
end
else
clone.Parent = unpackInto
end
end
else
warn(`Missing parent to unpack into for {curFolder}`)
end
end
unpackFolder(package, folder)
end;
local function CleanUp()
--local env = getfenv(2)
--local ran,ret = pcall(function() return env.script:GetFullName() end)
warn("Beginning Adonis cleanup & shutdown process...")
--warn(`CleanUp called from {tostring((ran and ret) or "Unknown")}`)
--local loader = server.Core.ClientLoader
server.Model.Name = "Adonis_Loader"
server.Model.Parent = service.ServerScriptService
server.Running = false
server.Logs.SaveCommandLogs()
server.Core.GAME_CLOSING = true;
server.Core.SaveAllPlayerData()
pcall(service.Threads.StopAll)
pcall(function()
for i, v in pairs(RbxEvents) do
print("Disconnecting event")
v:Disconnect()
table.remove(RbxEvents, i)
end
end)
--loader.Archivable = false
--loader.Disabled = true
--loader:Destroy()
if server.Core and server.Core.RemoteEvent then
pcall(server.Core.DisconnectEvent)
end
warn("Unloading complete")
end;
server = {
Running = true;
Modules = {};
Pcall = Pcall;
cPcall = cPcall;
Routine = Routine;
LogError = logError;
ErrorLogs = ErrorLogs;
ServerStartTime = os.time();
CommandCache = {};
};
locals = {
server = server;
CodeName = "";
Settings = server.Settings;
HookedEvents = HookedEvents;
ErrorLogs = ErrorLogs;
logError = logError;
origEnv = origEnv;
Routine = Routine;
Folder = Folder;
GetEnv = GetEnv;
cPcall = cPcall;
Pcall = Pcall;
};
service = require(Folder.Shared.Service)(function(eType, msg, desc, ...)
local extra = {...}
if eType == "MethodError" then
if server and server.Logs and server.Logs.AddLog then
server.Logs.AddLog("Script", {
Text = `Cached method doesn't match found method: {extra[1]}`;
Desc = `Method: {extra[1]}`
})
end
elseif eType == "ServerError" then
logError("Server", msg)
elseif eType == "TaskError" then
logError("Task", msg)
end
end, function(c, parent, tab)
if not isModule(c) and c ~= server.Loader and c ~= server.Dropper and c ~= server.Runner and c ~= server.Model and c ~= script and c ~= Folder and parent == nil then
tab.UnHook()
end
end, ServiceSpecific, GetEnv(nil, {server = server}))
|
--| Main |-- |
Remote.PhongDev.OnServerEvent:Connect(function(LocalPlayer)
local Humanoid = LocalPlayer.Character.Humanoid
Humanoid:LoadAnimation(Anims.Transform):Play() -- play animation
end)
|
-- Decompiled with the Synapse X Luau decompiler. |
local v1 = {};
local l__HDAdminMain__1 = _G.HDAdminMain;
function v1.GetRankName(p1, p2)
return l__HDAdminMain__1:GetModule("cf"):GetRankName(p2);
end;
function v1.GetRankId(p3, p4)
return l__HDAdminMain__1:GetModule("cf"):GetRankId(p4);
end;
function v1.Notice(p5, p6, p7)
if l__HDAdminMain__1.player then
if not p7 then
return;
end;
else
l__HDAdminMain__1:GetModule("cf"):Notice(p6, "Game Notice", p7);
return;
end;
l__HDAdminMain__1:GetModule("Notices"):Notice("Notice", "Game Notice", p7);
end;
function v1.Error(p8, p9, p10)
if l__HDAdminMain__1.player then
if not p10 then
return;
end;
else
l__HDAdminMain__1.signals.Error:FireClient(p9, { "Game Notice", p10 });
return;
end;
l__HDAdminMain__1:GetModule("Notices"):Notice("Error", "Game Notice", p10);
end;
return v1;
|
--[[
CameraModule - This ModuleScript implements a singleton class to manage the
selection, activation, and deactivation of the current camera controller,
character occlusion controller, and transparency controller. This script binds to
RenderStepped at Camera priority and calls the Update() methods on the active
controller instances.
The camera controller ModuleScripts implement classes which are instantiated and
activated as-needed, they are no longer all instantiated up front as they were in
the previous generation of PlayerScripts.
2018 PlayerScripts Update - AllYourBlox
--]] |
local CameraModule = {}
CameraModule.__index = CameraModule
local FFlagUserFlagEnableNewVRSystem do
local success, result = pcall(function()
return UserSettings():IsUserFeatureEnabled("UserFlagEnableNewVRSystem")
end)
FFlagUserFlagEnableNewVRSystem = success and result
end
|