crawl/depth
int64 0
2
| crawl/httpStatusCode
float64 200
200
⌀ | crawl/loadedTime
stringlengths 24
24
| crawl/loadedUrl
stringlengths 26
85
| crawl/referrerUrl
stringclasses 9
values | debug/requestHandlerMode
stringclasses 2
values | markdown
stringlengths 150
39.8k
| metadata/author
float64 | metadata/canonicalUrl
stringlengths 26
65
| metadata/description
stringlengths 8
428
⌀ | metadata/headers/access-control-allow-origin
stringclasses 1
value | metadata/headers/age
int64 0
0
| metadata/headers/alt-svc
stringclasses 1
value | metadata/headers/cache-control
stringclasses 1
value | metadata/headers/cf-cache-status
stringclasses 1
value | metadata/headers/cf-ray
stringlengths 20
20
| metadata/headers/connection
stringclasses 1
value | metadata/headers/content-encoding
stringclasses 1
value | metadata/headers/content-type
stringclasses 1
value | metadata/headers/date
stringlengths 29
29
| metadata/headers/expires
stringlengths 29
29
⌀ | metadata/headers/last-modified
stringclasses 2
values | metadata/headers/nel
stringclasses 2
values | metadata/headers/report-to
stringlengths 233
251
| metadata/headers/server
stringclasses 1
value | metadata/headers/transfer-encoding
stringclasses 1
value | metadata/headers/vary
stringclasses 1
value | metadata/headers/via
stringclasses 1
value | metadata/headers/x-cache
stringclasses 2
values | metadata/headers/x-cache-hits
int64 0
0
| metadata/headers/x-fastly-request-id
stringlengths 40
40
| metadata/headers/x-firefox-spdy
stringclasses 1
value | metadata/headers/x-github-request-id
stringlengths 30
36
| metadata/headers/x-origin-cache
stringclasses 1
value | metadata/headers/x-proxy-cache
stringclasses 1
value | metadata/headers/x-served-by
stringlengths 17
25
| metadata/headers/x-timer
stringlengths 26
30
| metadata/jsonLd
float64 | metadata/keywords
float64 | metadata/languageCode
stringclasses 1
value | metadata/openGraph/0/content
stringclasses 1
value | metadata/openGraph/0/property
stringclasses 1
value | metadata/openGraph/1/content
stringlengths 26
65
| metadata/openGraph/1/property
stringclasses 1
value | metadata/openGraph/2/content
stringlengths 12
38
| metadata/openGraph/2/property
stringclasses 1
value | metadata/openGraph/3/content
stringlengths 8
428
⌀ | metadata/openGraph/3/property
stringclasses 1
value | metadata/title
stringlengths 12
38
| screenshotUrl
float64 | text
stringlengths 108
34.1k
| url
stringlengths 26
85
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 200 | 2024-04-23T15:11:06.208Z | https://wasp-lang.dev/docs | https://wasp-lang.dev/docs | browser | ## Introduction
note
If you are looking for the installation instructions, check out the [Quick Start](https://wasp-lang.dev/docs/quick-start) section.
We will give a brief overview of what Wasp is, how it works on a high level and when to use it.
It is an opinionated way of building **full-stack web applications**. It takes care of all three major parts of a web application: **client** (front-end), **server** (back-end) and **database**.
### Works well with your existing stack[](#works-well-with-your-existing-stack "Direct link to Works well with your existing stack")
Wasp is not trying to do everything at once but rather focuses on the complexity which arises from connecting all the parts of the stack (client, server, database, deployment) together.
Wasp is using **React**, **Node.js** and **Prisma** under the hood and relies on them to define web components and server queries and actions.
### Wasp's secret sauce[](#wasps-secret-sauce "Direct link to Wasp's secret sauce")
At the core is the Wasp compiler which takes the Wasp config and your Javascript code and outputs the client app, server app and deployment code.
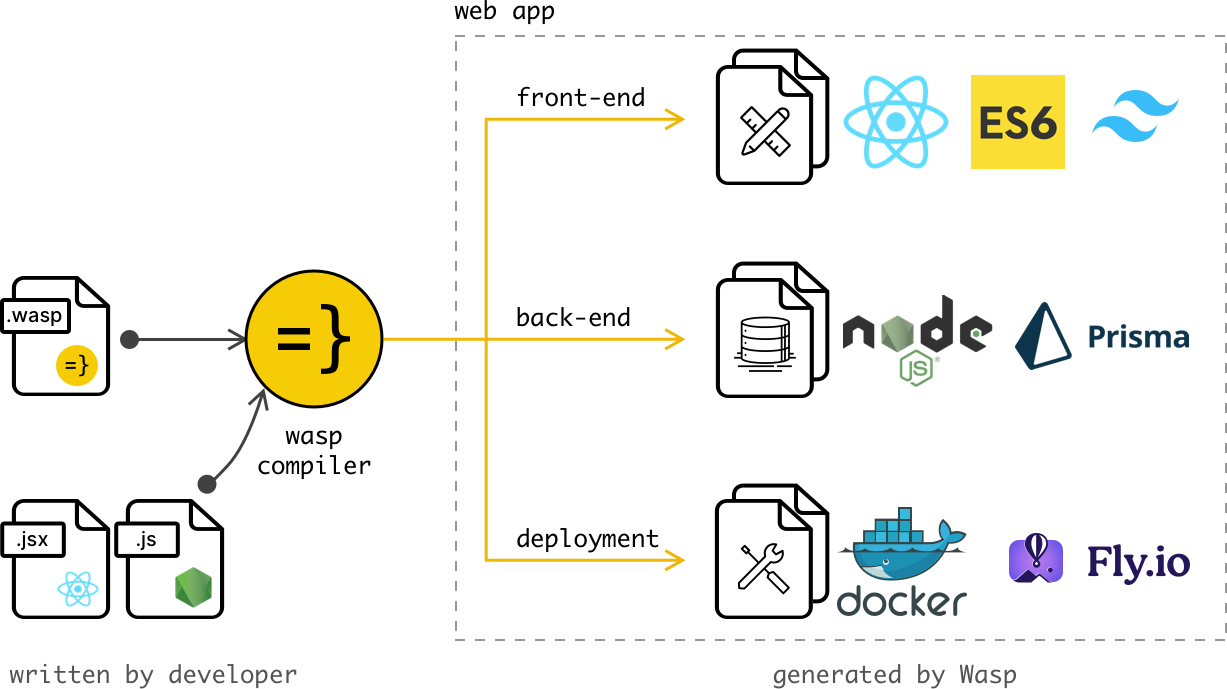
How the magic happens 🌈
The cool thing about having a compiler that understands your code is that it can do a lot of things for you.
Define your app in the Wasp config and get:
* login and signup with Auth UI components,
* full-stack type safety,
* e-mail sending,
* async processing jobs,
* React Query powered data fetching,
* security best practices,
* and more.
You don't need to write any code for these features, Wasp will take care of it for you 🤯 And what's even better, Wasp also maintains the code for you, so you don't have to worry about keeping up with the latest security best practices. As Wasp updates, so does your app.
## So what does the code look like?[](#so-what-does-the-code-look-like "Direct link to So what does the code look like?")
Let's say you want to build a web app that allows users to **create and share their favorite recipes**.
Let's start with the main.wasp file: it is the central file of your app, where you describe the app from the high level.
Let's give our app a title and let's immediately turn on the full-stack authentication via username and password:
main.wasp
```
app RecipeApp { title: "My Recipes", wasp: { version: "^0.13.0" }, auth: { methods: { usernameAndPassword: {} }, onAuthFailedRedirectTo: "/login", userEntity: User }}
```
Let's then add the data models for your recipes. We will want to have Users and Users can own Recipes:
main.wasp
```
...entity User {=psl // Data models are defined using Prisma Schema Language. id Int @id @default(autoincrement()) recipes Recipe[]psl=}entity Recipe {=psl id Int @id @default(autoincrement()) title String description String? userId Int user User @relation(fields: [userId], references: [id])psl=}
```
Next, let's define how to do something with these data models!
We do that by defining Operations, in this case a Query `getRecipes` and Action `addRecipe`, which are in their essence a Node.js functions that execute on server and can, thanks to Wasp, very easily be called from the client.
First, we define these Operations in our main.wasp file, so Wasp knows about them and can "beef them up":
main.wasp
```
// Queries have automatic cache invalidation and are type-safe.query getRecipes { fn: import { getRecipes } from "@src/recipe/operations.ts", entities: [Recipe],}// Actions are type-safe and can be used to perform side-effects.action addRecipe { fn: import { addRecipe } from "@src/recipe/operations.ts", entities: [Recipe],}
```
... and then implement them in our Javascript (or TypeScript) code (we show just the query here, using TypeScript):
src/recipe/operations.ts
```
// Wasp generates the types for you.import { type GetRecipes } from "wasp/server/operations";import { type Recipe } from "wasp/entities";export const getRecipes: GetRecipes<{}, Recipe[]> = async (_args, context) => { return context.entities.Recipe.findMany( // Prisma query { where: { user: { id: context.user.id } } } );};export const addRecipe ...
```
Now we can very easily use these in our React components!
For the end, let's create a home page of our app.
First we define it in main.wasp:
main.wasp
```
...route HomeRoute { path: "/", to: HomePage }page HomePage { component: import { HomePage } from "@src/pages/HomePage", authRequired: true // Will send user to /login if not authenticated.}
```
and then implement it as a React component in JS/TS (that calls the Operations we previously defined):
src/pages/HomePage.tsx
```
import { useQuery, getRecipes } from "wasp/client/operations";import { type User } from "wasp/entities";export function HomePage({ user }: { user: User }) { // Due to full-stack type safety, `recipes` will be of type `Recipe[]` here. const { data: recipes, isLoading } = useQuery(getRecipes); // Calling our query here! if (isLoading) { return <div>Loading...</div>; } return ( <div> <h1>Recipes</h1> <ul> {recipes ? recipes.map((recipe) => ( <li key={recipe.id}> <div>{recipe.title}</div> <div>{recipe.description}</div> </li> )) : 'No recipes defined yet!'} </ul> </div> );}
```
And voila! We are listing all the recipes in our app 🎉
This was just a quick example to give you a taste of what Wasp is. For step by step tour through the most important Wasp features, check out the [Todo app tutorial](https://wasp-lang.dev/docs/tutorial/create).
note
Above we skipped defining `/login` and `/signup` pages to keep the example a bit shorter, but those are very simple to do by using Wasp's Auth UI feature.
## When to use Wasp[](#when-to-use-wasp "Direct link to When to use Wasp")
Wasp is addressing the same core problems that typical web app frameworks are addressing, and it in big part [looks, swims and quacks](https://en.wikipedia.org/wiki/Duck_test) like a web app framework.
### Best used for[](#best-used-for "Direct link to Best used for")
* building full-stack web apps (like e.g. Airbnb or Asana)
* quickly starting a web app with industry best practices
* to be used alongside modern web dev stack (currently supported React and Node)
### Avoid using Wasp for[](#avoid-using-wasp-for "Direct link to Avoid using Wasp for")
* building static/presentational websites
* to be used as a no-code solution
* to be a solve-it-all tool in a single language
## Wasp is a DSL[](#wasp-is-a-dsl "Direct link to Wasp is a DSL")
note
You don't need to know what a DSL is to use Wasp, but if you are curious, you can read more about it below.
Wasp does not match typical expectations of a web app framework: it is not a set of libraries, it is instead a simple programming language that understands your code and can do a lot of things for you.
Wasp is a programming language, but a specific kind: it is specialized for a single purpose: **building modern web applications**. We call such languages _DSL_s (Domain Specific Language).
Other examples of _DSL_s that are often used today are e.g. _SQL_ for databases and _HTML_ for web page layouts. The main advantage and reason why _DSL_s exist is that they need to do only one task (e.g. database queries) so they can do it well and provide the best possible experience for the developer.
The same idea stands behind Wasp - a language that will allow developers to **build modern web applications with 10x less code and less stack-specific knowledge**. | null | https://wasp-lang.dev/docs | If you are looking for the installation instructions, check out the Quick Start section. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ec8a35d714bcf-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:11:01 GMT | Tue, 23 Apr 2024 15:21:01 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=ZT0IS6bdPIlLUYwJa4kd8wIiwZSLdenKtJS9kvs289oSRofo%2BSthoBpIdWkOzdNXBVMWSiWZIl31ESbvIMmn9DfgWYGoAGRK6YGdKMGF8jsAOkrqsup%2BSEsNDC3czSnX"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | ad08172eddb5041ed557cdd511b3744770b26a99 | h2 | 8212:180046:3C22E64:4727758:6627CF85 | null | MISS | cache-nyc-kteb1890025-NYC | S1713885062.689630, VS0, VE21 | null | null | en | og:image | https://wasp-lang.dev/docs | og:url | Introduction | Wasp | og:title | If you are looking for the installation instructions, check out the Quick Start section. | og:description | Introduction | Wasp | null | Introduction
note
If you are looking for the installation instructions, check out the Quick Start section.
We will give a brief overview of what Wasp is, how it works on a high level and when to use it.
It is an opinionated way of building full-stack web applications. It takes care of all three major parts of a web application: client (front-end), server (back-end) and database.
Works well with your existing stack
Wasp is not trying to do everything at once but rather focuses on the complexity which arises from connecting all the parts of the stack (client, server, database, deployment) together.
Wasp is using React, Node.js and Prisma under the hood and relies on them to define web components and server queries and actions.
Wasp's secret sauce
At the core is the Wasp compiler which takes the Wasp config and your Javascript code and outputs the client app, server app and deployment code.
How the magic happens 🌈
The cool thing about having a compiler that understands your code is that it can do a lot of things for you.
Define your app in the Wasp config and get:
login and signup with Auth UI components,
full-stack type safety,
e-mail sending,
async processing jobs,
React Query powered data fetching,
security best practices,
and more.
You don't need to write any code for these features, Wasp will take care of it for you 🤯 And what's even better, Wasp also maintains the code for you, so you don't have to worry about keeping up with the latest security best practices. As Wasp updates, so does your app.
So what does the code look like?
Let's say you want to build a web app that allows users to create and share their favorite recipes.
Let's start with the main.wasp file: it is the central file of your app, where you describe the app from the high level.
Let's give our app a title and let's immediately turn on the full-stack authentication via username and password:
main.wasp
app RecipeApp {
title: "My Recipes",
wasp: { version: "^0.13.0" },
auth: {
methods: { usernameAndPassword: {} },
onAuthFailedRedirectTo: "/login",
userEntity: User
}
}
Let's then add the data models for your recipes. We will want to have Users and Users can own Recipes:
main.wasp
...
entity User {=psl // Data models are defined using Prisma Schema Language.
id Int @id @default(autoincrement())
recipes Recipe[]
psl=}
entity Recipe {=psl
id Int @id @default(autoincrement())
title String
description String?
userId Int
user User @relation(fields: [userId], references: [id])
psl=}
Next, let's define how to do something with these data models!
We do that by defining Operations, in this case a Query getRecipes and Action addRecipe, which are in their essence a Node.js functions that execute on server and can, thanks to Wasp, very easily be called from the client.
First, we define these Operations in our main.wasp file, so Wasp knows about them and can "beef them up":
main.wasp
// Queries have automatic cache invalidation and are type-safe.
query getRecipes {
fn: import { getRecipes } from "@src/recipe/operations.ts",
entities: [Recipe],
}
// Actions are type-safe and can be used to perform side-effects.
action addRecipe {
fn: import { addRecipe } from "@src/recipe/operations.ts",
entities: [Recipe],
}
... and then implement them in our Javascript (or TypeScript) code (we show just the query here, using TypeScript):
src/recipe/operations.ts
// Wasp generates the types for you.
import { type GetRecipes } from "wasp/server/operations";
import { type Recipe } from "wasp/entities";
export const getRecipes: GetRecipes<{}, Recipe[]> = async (_args, context) => {
return context.entities.Recipe.findMany( // Prisma query
{ where: { user: { id: context.user.id } } }
);
};
export const addRecipe ...
Now we can very easily use these in our React components!
For the end, let's create a home page of our app.
First we define it in main.wasp:
main.wasp
...
route HomeRoute { path: "/", to: HomePage }
page HomePage {
component: import { HomePage } from "@src/pages/HomePage",
authRequired: true // Will send user to /login if not authenticated.
}
and then implement it as a React component in JS/TS (that calls the Operations we previously defined):
src/pages/HomePage.tsx
import { useQuery, getRecipes } from "wasp/client/operations";
import { type User } from "wasp/entities";
export function HomePage({ user }: { user: User }) {
// Due to full-stack type safety, `recipes` will be of type `Recipe[]` here.
const { data: recipes, isLoading } = useQuery(getRecipes); // Calling our query here!
if (isLoading) {
return <div>Loading...</div>;
}
return (
<div>
<h1>Recipes</h1>
<ul>
{recipes ? recipes.map((recipe) => (
<li key={recipe.id}>
<div>{recipe.title}</div>
<div>{recipe.description}</div>
</li>
)) : 'No recipes defined yet!'}
</ul>
</div>
);
}
And voila! We are listing all the recipes in our app 🎉
This was just a quick example to give you a taste of what Wasp is. For step by step tour through the most important Wasp features, check out the Todo app tutorial.
note
Above we skipped defining /login and /signup pages to keep the example a bit shorter, but those are very simple to do by using Wasp's Auth UI feature.
When to use Wasp
Wasp is addressing the same core problems that typical web app frameworks are addressing, and it in big part looks, swims and quacks like a web app framework.
Best used for
building full-stack web apps (like e.g. Airbnb or Asana)
quickly starting a web app with industry best practices
to be used alongside modern web dev stack (currently supported React and Node)
Avoid using Wasp for
building static/presentational websites
to be used as a no-code solution
to be a solve-it-all tool in a single language
Wasp is a DSL
note
You don't need to know what a DSL is to use Wasp, but if you are curious, you can read more about it below.
Wasp does not match typical expectations of a web app framework: it is not a set of libraries, it is instead a simple programming language that understands your code and can do a lot of things for you.
Wasp is a programming language, but a specific kind: it is specialized for a single purpose: building modern web applications. We call such languages DSLs (Domain Specific Language).
Other examples of DSLs that are often used today are e.g. SQL for databases and HTML for web page layouts. The main advantage and reason why DSLs exist is that they need to do only one task (e.g. database queries) so they can do it well and provide the best possible experience for the developer.
The same idea stands behind Wasp - a language that will allow developers to build modern web applications with 10x less code and less stack-specific knowledge. | https://wasp-lang.dev/docs |
|
1 | 200 | 2024-04-23T15:11:22.222Z | https://wasp-lang.dev/docs/vision | https://wasp-lang.dev/docs | browser | ## Vision
With Wasp, we want to make developing web apps easy and enjoyable, for novices and experts in web development alike.
Ideal we are striving for is that programming in Wasp feels like describing an app using a human language - like writing a specification document where you describe primarily your requirements and as little implementation details as possible. Creating a new production-ready web app should be easy and deploying it to production should be straightforward.
That is why we believe Wasp needs to be a programming language (DSL) and not a library - we want to capture all parts of the web app into one integrated system that is perfectly tailored just for that purpose.
On the other hand, we believe that trying to capture every single detail in one language would not be reasonable. There are solutions out there that work very well for the specific task they aim to solve (React for web components, CSS/HTML for design/markup, JS/TS for logic, ...) and we don't want to replace them with Wasp. Instead, we see Wasp as a declarative "glue" code uniting all these specific solutions and providing a higher-level notion of the web app above them.
Wasp is still early in its development and therefore far from where we imagine it will be in the future. This is what we imagine:
* **Declarative, static language** with simple basic rules and **that understands a lot of web app concepts** - "horizontal language". Supports multiple files/modules, libraries.
* **Integrates seamlessly with the most popular technologies** for building specific, more complex parts of the web app (React, CSS, JS, ...). They can be used inline (mixed with Wasp code) or provided via external files.
* **Has hatches (escape mechanisms) that allow you to customize your web app** in all the right places, but remain hidden until you need them.
* **Entity (data model) is a first-class citizen** - defined via custom Wasp syntax and it integrates very closely with the rest of the features, serving as one of the central concepts around which everything is built.
* **Out of the box** support for CRUD UI based on the Entities, to get you quickly going, but also customizable to some level.
* **"Smart" operations (queries and actions)** that in most cases automatically figure out when to update, and if not it is easy to define custom logic to compensate for that. User worries about client-server gap as little as possible.
* Support, directly in Wasp, for **declaratively defining simple components and operations**.
* Besides Wasp as a programming language, there will also be a **visual builder that generates/edits Wasp code**, allowing non-developers to participate in development. Since Wasp is declarative, we imagine such builder to naturally follow from Wasp language.
* **Server side rendering, caching, packaging, security**, ... -> all those are taken care of by Wasp. You tell Wasp what you want, and Wasp figures out how to do it.
* As **simple deployment to production/staging** as it gets.
* While it comes with the official implementation(s), **Wasp language will not be coupled with the single implementation**. Others can provide implementations that compile to different web app stacks. | null | https://wasp-lang.dev/docs/vision | With Wasp, we want to make developing web apps easy and enjoyable, for novices and experts in web development alike. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ec9007ae64bcf-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:11:16 GMT | Tue, 23 Apr 2024 15:21:16 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=X3S8t1YhmncwAhc4B%2FyLSjhBUM8QH53eKgLdG1FZ3MB3vigATHKpYFw26TFI%2Foh2300atl8m62i0FnLtpqQ7m9IoOXAEK4Qv98%2FjZrpc9lXmOZz6IacJIFh575FnmPT1"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 56a4b10c9295f5795426b484d4660acea1deee3a | h2 | D3FC:209F3A:3A75104:4579FBA:6627CF93 | HIT | MISS | cache-nyc-kteb1890025-NYC | S1713885077.567829, VS0, VE17 | null | null | en | og:image | https://wasp-lang.dev/docs/vision | og:url | Vision | Wasp | og:title | With Wasp, we want to make developing web apps easy and enjoyable, for novices and experts in web development alike. | og:description | Vision | Wasp | null | Vision
With Wasp, we want to make developing web apps easy and enjoyable, for novices and experts in web development alike.
Ideal we are striving for is that programming in Wasp feels like describing an app using a human language - like writing a specification document where you describe primarily your requirements and as little implementation details as possible. Creating a new production-ready web app should be easy and deploying it to production should be straightforward.
That is why we believe Wasp needs to be a programming language (DSL) and not a library - we want to capture all parts of the web app into one integrated system that is perfectly tailored just for that purpose.
On the other hand, we believe that trying to capture every single detail in one language would not be reasonable. There are solutions out there that work very well for the specific task they aim to solve (React for web components, CSS/HTML for design/markup, JS/TS for logic, ...) and we don't want to replace them with Wasp. Instead, we see Wasp as a declarative "glue" code uniting all these specific solutions and providing a higher-level notion of the web app above them.
Wasp is still early in its development and therefore far from where we imagine it will be in the future. This is what we imagine:
Declarative, static language with simple basic rules and that understands a lot of web app concepts - "horizontal language". Supports multiple files/modules, libraries.
Integrates seamlessly with the most popular technologies for building specific, more complex parts of the web app (React, CSS, JS, ...). They can be used inline (mixed with Wasp code) or provided via external files.
Has hatches (escape mechanisms) that allow you to customize your web app in all the right places, but remain hidden until you need them.
Entity (data model) is a first-class citizen - defined via custom Wasp syntax and it integrates very closely with the rest of the features, serving as one of the central concepts around which everything is built.
Out of the box support for CRUD UI based on the Entities, to get you quickly going, but also customizable to some level.
"Smart" operations (queries and actions) that in most cases automatically figure out when to update, and if not it is easy to define custom logic to compensate for that. User worries about client-server gap as little as possible.
Support, directly in Wasp, for declaratively defining simple components and operations.
Besides Wasp as a programming language, there will also be a visual builder that generates/edits Wasp code, allowing non-developers to participate in development. Since Wasp is declarative, we imagine such builder to naturally follow from Wasp language.
Server side rendering, caching, packaging, security, ... -> all those are taken care of by Wasp. You tell Wasp what you want, and Wasp figures out how to do it.
As simple deployment to production/staging as it gets.
While it comes with the official implementation(s), Wasp language will not be coupled with the single implementation. Others can provide implementations that compile to different web app stacks. | https://wasp-lang.dev/docs/vision |
|
1 | 200 | 2024-04-23T15:11:18.440Z | https://wasp-lang.dev/docs/contributing | https://wasp-lang.dev/docs | browser | Version: 0.13.0
## Contributing
Any way you want to contribute is a good way, and we'd be happy to meet you! A single entry point for all contributors is the [CONTRIBUTING.md](https://github.com/wasp-lang/wasp/blob/main/CONTRIBUTING.md) file in our Github repo. All the requirements and instructions are there, so please check [CONTRIBUTING.md](https://github.com/wasp-lang/wasp/blob/main/CONTRIBUTING.md) for more details.
Some side notes to make your journey easier:
1. Join us on [Discord](https://discord.gg/rzdnErX) and let's talk! We can discuss language design, new/existing features, and weather, or you can tell us how you feel about Wasp :).
2. Wasp's compiler is built with Haskell. That means you'll need to be somewhat familiar with this language if you'd like to contribute to the compiler itself. But Haskell is just a part of Wasp, and you can contribute to lot of parts that require web dev skills, either by coding or by suggesting how to improve Wasp and its design as a web framework. If you don't have Haskell knowledge (or any dev experience at all) - no problem. There are a lot of JS-related tasks and documentation updates as well!
3. If there's something you'd like to bring to our attention, go to [docs GitHub repo](https://github.com/wasp-lang/wasp) and make an issue/PR!
Happy hacking! | null | https://wasp-lang.dev/docs/contributing | Any way you want to contribute is a good way, and we'd be happy to meet you! A single entry point for all contributors is the CONTRIBUTING.md file in our Github repo. All the requirements and instructions are there, so please check CONTRIBUTING.md for more details. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ec8f5598f4bcf-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:11:14 GMT | Tue, 23 Apr 2024 15:21:14 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=vA7z7gSIGyAqYTW7qe6S%2BB4NwIB%2FPda5rsVlLYKq%2BpCfdC9dSYnkcM27MmkU%2BfGAwnDh1WO0KVdRDKnd%2FpjeWASxhW9wrP%2Fx5LPAbqFktARMNBiCDUhvTCN0hqTIvMJt"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 240de283f418bfc4db77d984ea9bb38cb31054a0 | h2 | 68AA:170D:256FDC:2CE104:6627CF92 | null | MISS | cache-nyc-kteb1890025-NYC | S1713885075.788575, VS0, VE20 | null | null | en | og:image | https://wasp-lang.dev/docs/contributing | og:url | Contributing | Wasp | og:title | Any way you want to contribute is a good way, and we'd be happy to meet you! A single entry point for all contributors is the CONTRIBUTING.md file in our Github repo. All the requirements and instructions are there, so please check CONTRIBUTING.md for more details. | og:description | Contributing | Wasp | null | Version: 0.13.0
Contributing
Any way you want to contribute is a good way, and we'd be happy to meet you! A single entry point for all contributors is the CONTRIBUTING.md file in our Github repo. All the requirements and instructions are there, so please check CONTRIBUTING.md for more details.
Some side notes to make your journey easier:
Join us on Discord and let's talk! We can discuss language design, new/existing features, and weather, or you can tell us how you feel about Wasp :).
Wasp's compiler is built with Haskell. That means you'll need to be somewhat familiar with this language if you'd like to contribute to the compiler itself. But Haskell is just a part of Wasp, and you can contribute to lot of parts that require web dev skills, either by coding or by suggesting how to improve Wasp and its design as a web framework. If you don't have Haskell knowledge (or any dev experience at all) - no problem. There are a lot of JS-related tasks and documentation updates as well!
If there's something you'd like to bring to our attention, go to docs GitHub repo and make an issue/PR!
Happy hacking! | https://wasp-lang.dev/docs/contributing |
|
1 | 200 | 2024-04-23T15:11:24.759Z | https://wasp-lang.dev/docs/telemetry | https://wasp-lang.dev/docs | browser | ```
{ // Randomly generated, non-identifiable UUID representing a user. "distinct_id": "bf3fa7a8-1c11-4f82-9542-ec1a2d28786b", // Non-identifiable hash representing a project. "project_hash": "6d7e561d62b955d1", // True if command was `wasp build`, false otherwise. "is_build": true, // Captures `wasp deploy ...` args, but only those from the limited, pre-defined list of keywords. // Those are "fly", "setup", "create-db", "deploy" and "cmd". Everything else is ommited. "deploy_cmd_args": "fly;deploy", "wasp_version": "0.1.9.1", "os": "linux", // "CI" if running on CI, and whatever is the content of "WASP_TELEMETRY_CONTEXT" env var. // We use this to track when execution is happening in some special context, like on Gitpod, Replit or similar. "context": "CI"}
``` | null | https://wasp-lang.dev/docs/telemetry | Overview | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ec90c5d454bcf-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:11:18 GMT | Tue, 23 Apr 2024 15:21:18 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=asaNfjrmWg%2Ftfptie69yCS2GKJ4%2Fbg57cEyoWNHm8WggbcvI2qDOseQMQT%2Bi6LWJ4iyE6dMu5f4J5cK8pIPVZBwWpyTc2dwXGqrDVZ0E6KZNuwXGmyEsM9mT%2FPM3PhDa"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 7fecc340551b85a2b8cb9462f1f48fdb134e58bd | h2 | EBAC:173D:D0C89:FF012:6627CF95 | HIT | MISS | cache-nyc-kteb1890025-NYC | S1713885078.463631, VS0, VE16 | null | null | en | og:image | https://wasp-lang.dev/docs/telemetry | og:url | Telemetry | Wasp | og:title | Overview | og:description | Telemetry | Wasp | null | {
// Randomly generated, non-identifiable UUID representing a user.
"distinct_id": "bf3fa7a8-1c11-4f82-9542-ec1a2d28786b",
// Non-identifiable hash representing a project.
"project_hash": "6d7e561d62b955d1",
// True if command was `wasp build`, false otherwise.
"is_build": true,
// Captures `wasp deploy ...` args, but only those from the limited, pre-defined list of keywords.
// Those are "fly", "setup", "create-db", "deploy" and "cmd". Everything else is ommited.
"deploy_cmd_args": "fly;deploy",
"wasp_version": "0.1.9.1",
"os": "linux",
// "CI" if running on CI, and whatever is the content of "WASP_TELEMETRY_CONTEXT" env var.
// We use this to track when execution is happening in some special context, like on Gitpod, Replit or similar.
"context": "CI"
} | https://wasp-lang.dev/docs/telemetry |
|
1 | 200 | 2024-04-23T15:11:25.654Z | https://wasp-lang.dev/docs/contact | https://wasp-lang.dev/docs | browser | Version: 0.13.0
## Contact
You can find us on [Discord](https://discord.gg/rzdnErX) or you can reach out to us via email at [hi@wasp-lang.dev](mailto:hi@wasp-lang.dev). | null | https://wasp-lang.dev/docs/contact | You can find us on Discord or you can reach out to us via email at hi@wasp-lang.dev. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ec91899614bcf-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:11:20 GMT | Tue, 23 Apr 2024 15:21:20 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=4GBkPSB08KYFE9NLLPILsB8hiyu85dZ5%2Fkpe97BMIcsn4aRxbXsQWBx6ntFeFUZ6VherzW29iD78gWQ58gzMEa%2FEZCC0QNDIiFBvXoHyeBgLp8w7YC4APjT9ZWX19TB0"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 427b9cb0d86b78a28ada0a2b8f6338326878f006 | h2 | 7002:1055D1:3A693B6:456D4BF:6627CF98 | HIT | MISS | cache-nyc-kteb1890025-NYC | S1713885080.429227, VS0, VE30 | null | null | en | og:image | https://wasp-lang.dev/docs/contact | og:url | Contact | Wasp | og:title | You can find us on Discord or you can reach out to us via email at hi@wasp-lang.dev. | og:description | Contact | Wasp | null | Version: 0.13.0
Contact
You can find us on Discord or you can reach out to us via email at hi@wasp-lang.dev. | https://wasp-lang.dev/docs/contact |
|
1 | 200 | 2024-04-23T15:11:38.420Z | https://wasp-lang.dev/docs/migrate-from-0-11-to-0-12 | https://wasp-lang.dev/docs | browser | The latest version of Wasp is 0.13.X
To fully migrate from 0.11.X to the latest version of Wasp, you should first migrate to **0.12.X and then to 0.13.X**.
Make sure to read the [migration guide from 0.12.X to 0.13.X](https://wasp-lang.dev/docs/migrate-from-0-12-to-0-13) after you finish this one.
## What's new in Wasp 0.12.0?[](#whats-new-in-wasp-0120 "Direct link to What's new in Wasp 0.12.0?")
### New project structure[](#new-project-structure "Direct link to New project structure")
Here's a file tree of a fresh Wasp project created with the previous version of Wasp. More precisely, this is what you'll get if you run `wasp new myProject` using Wasp 0.11.x:
```
.├── .gitignore├── main.wasp├── src│ ├── client│ │ ├── Main.css│ │ ├── MainPage.jsx│ │ ├── react-app-env.d.ts│ │ ├── tsconfig.json│ │ └── waspLogo.png│ ├── server│ │ └── tsconfig.json│ ├── shared│ │ └── tsconfig.json│ └── .waspignore└── .wasproot
```
Compare that with the file tree of a fresh Wasp project created with Wasp 0.12.0. In other words, this is what you will get by running `wasp new myProject` from this point onwards:
```
.├── .gitignore├── main.wasp├── package.json├── public│ └── .gitkeep├── src│ ├── Main.css│ ├── MainPage.jsx│ ├── queries.ts│ ├── vite-env.d.ts│ ├── .waspignore│ └── waspLogo.png├── tsconfig.json├── vite.config.ts└── .wasproot
```
The main differences are:
* The server/client code separation is no longer necessary. You can now organize your code however you want, as long as it's inside the `src` directory.
* All external imports in your Wasp file must have paths starting with `@src` (e.g., `import foo from '@src/bar.js'`) where `@src` refers to the `src` directory in your project root. The paths can no longer start with `@server` or `@client`.
* Your project now features a top-level `public` dir. Wasp will publicly serve all the files it finds in this directory. Read more about it [here](https://wasp-lang.dev/docs/project/static-assets).
Our [Overview docs](https://wasp-lang.dev/docs/tutorial/project-structure) explain the new structure in detail, while this page provides a [quick guide](#migrating-your-project-to-the-new-structure) for migrating existing projects.
### New auth[](#new-auth "Direct link to New auth")
In Wasp 0.11.X, authentication was based on the `User` model which the developer needed to set up properly and take care of the auth fields like `email` or `password`.
main.wasp
```
app myApp { wasp: { version: "^0.11.0" }, title: "My App", auth: { userEntity: User, externalAuthEntity: SocialLogin, methods: { gitHub: {} }, onAuthFailedRedirectTo: "/login" },}entity User {=psl id Int @id @default(autoincrement()) username String @unique password String externalAuthAssociations SocialLogin[]psl=}entity SocialLogin {=psl id Int @id @default(autoincrement()) provider String providerId String user User @relation(fields: [userId], references: [id], onDelete: Cascade) userId Int createdAt DateTime @default(now()) @@unique([provider, providerId, userId])psl=}
```
From 0.12.X onwards, authentication is based on the auth models which are automatically set up by Wasp. You don't need to take care of the auth fields anymore.
The `User` model is now just a business logic model and you use it for storing the data that is relevant for your app.
main.wasp
```
app myApp { wasp: { version: "^0.12.0" }, title: "My App", auth: { userEntity: User, methods: { gitHub: {} }, onAuthFailedRedirectTo: "/login" },}entity User {=psl id Int @id @default(autoincrement())psl=}
```
Regression Note: Multiple Auth Identities per User
With our old auth implementation, if you were using both Google and email auth methods, your users could sign up with Google first and then, later on, reset their password and therefore also enable logging in with their email and password. This was the only way in which a single user could have multiple login methods at the same time (Google and email).
This is not possible anymore. **The new auth system doesn't support multiple login methods per user at the moment**. We do plan to add this soon though, with the introduction of the [account merging feature](https://github.com/wasp-lang/wasp/issues/954).
If you have any users that have both Google and email login credentials at the same time, you will have to pick only one of those for that user to keep when migrating them.
Regression Note: `_waspCustomValidations` is deprecated
Auth field customization is no longer possible using the `_waspCustomValidations` on the `User` entity. This is a part of auth refactoring that we are doing to make it easier to customize auth. We will be adding more customization options in the future.
You can read more about the new auth system in the [Auth Entities](https://wasp-lang.dev/docs/auth/entities) section.
## How to Migrate?[](#how-to-migrate "Direct link to How to Migrate?")
These instructions are for migrating your app from Wasp `0.11.X` to Wasp `0.12.X`, meaning they will work for all minor releases that fit this pattern (e.g., the guide applies to `0.12.0`, `0.12.1`, ...).
The guide consists of two big steps:
1. Migrating your Wasp project to the new structure.
2. Migrating to the new auth.
If you get stuck at any point, don't hesitate to ask for help on [our Discord server](https://discord.gg/rzdnErX).
### Migrating Your Project to the New Structure[](#migrating-your-project-to-the-new-structure "Direct link to Migrating Your Project to the New Structure")
You can easily migrate your old Wasp project to the new structure by following a series of steps. Assuming you have a project called `foo` inside the directory `foo`, you should:
0. **Install the `0.12.x` version** of Wasp.
```
curl -sSL https://get.wasp-lang.dev/installer.sh | sh -s -- -v 0.12.4
```
1. Make sure to **backup or save your project** before starting the procedure (e.g., by committing it to source control or creating a copy).
2. **Position yourself in the terminal** in the directory that is a parent of your wasp project directory (so one level above: if you do `ls`, you should see your wasp project dir listed).
3. **Run the migration script** (replace `foo` at the end with the name of your Wasp project directory) and follow the instructions:
In case the migration script doesn't work well for you, you can do the same steps manually, as described here:
That's it! You now have a properly structured Wasp 0.12.0 project in the `foo` directory. Your app probably doesn't quite work yet due to some other changes in Wasp 0.12.0, but we'll get to that in the next sections.
### Migrating declaration names[](#migrating-declaration-names "Direct link to Migrating declaration names")
Wasp 0.12.0 adds a casing constraints when naming Queries, Actions, Jobs, and Entities in the `main.wasp` file.
The following casing conventions have now become mandatory:
* Operation (i.e., Query and Action) names must begin with a lowercase letter: `query getTasks {...}`, `action createTask {...}`.
* Job names must begin with a lowercase letter: `job sendReport {...}`.
* Entity names must start with an uppercase letter: `entity Task {...}`.
### Migrating the Tailwind Setup[](#migrating-the-tailwind-setup "Direct link to Migrating the Tailwind Setup")
note
If you don't use Tailwind in your project, you can skip this section.
There is a small change in how the `tailwind.config.cjs` needs to be defined in Wasp 0.12.0.
You'll need to wrap all your paths in the `content` field with the `resolveProjectPath` function. This makes sure that the paths are resolved correctly when generating your CSS.
Here's how you can do it:
* Before
* After
tailwind.config.cjs
```
/** @type {import('tailwindcss').Config} */module.exports = { content: [ './src/**/*.{js,jsx,ts,tsx}', ], theme: { extend: {}, }, plugins: [],}
```
### Default Server Dockerfile Changed[](#default-server-dockerfile-changed "Direct link to Default Server Dockerfile Changed")
note
If you didn't customize your Dockerfile or had a custom build process for the Wasp server, you can skip this section.
Between Wasp 0.11.X and 0.12.X, the Dockerfile that Wasp generates for you for deploying the server has changed. If you defined a custom Dockerfile in your project root dir or in any other way relied on its contents, you'll need to update it to incorporate the changes that Wasp 0.12.X made.
We suggest that you temporarily move your custom Dockerfile to a different location, then run `wasp start` to generate the new Dockerfile. Check out the `.wasp/out/Dockerfile` to see the new Dockerfile and what changes you need to make. You'll probably need to copy some of the changes from the new Dockerfile to your custom one to make your app work with Wasp 0.12.X.
### Migrating to the New Auth[](#migrating-to-the-new-auth "Direct link to Migrating to the New Auth")
As shown in [the previous section](#new-auth), Wasp significantly changed how authentication works in version 0.12.0. This section leads you through migrating your app from Wasp 0.11.X to Wasp 0.12.X.
Migrating your existing app to the new auth system is a two-step process:
1. Migrate to the new auth system
2. Clean up the old auth system
Migrating a deployed app
While going through these steps, we will focus first on doing the changes locally (including your local development database).
Once we confirm everything works well locally, we will apply the same changes to the deployed app (including your production database).
**We'll put extra info for migrating a deployed app in a box like this one.**
#### 1\. Migrate to the New Auth System[](#1-migrate-to-the-new-auth-system "Direct link to 1. Migrate to the New Auth System")
You can follow these steps to migrate to the new auth system (assuming you already migrated the project structure to 0.12, as described [above](#migrating-your-project-to-the-new-structure)):
1. **Migrate `getUserFields` and/or `additionalSignupFields` in the `main.wasp` file to the new `userSignupFields` field.**
If you are not using them, you can skip this step.
In Wasp 0.11.X, you could define a `getUserFieldsFn` to specify extra fields that would get saved to the `User` when using Google or GitHub to sign up.
You could also define `additionalSignupFields` to specify extra fields for the Email or Username & Password signup.
In 0.12.X, we unified these two concepts into the `userSignupFields` field.
Migration for [Email](https://wasp-lang.dev/docs/auth/email) and [Username & Password](https://wasp-lang.dev/docs/auth/username-and-pass)Migration for [Github](https://wasp-lang.dev/docs/auth/social-auth/github) and [Google](https://wasp-lang.dev/docs/auth/social-auth/google)
2. **Remove the `auth.methods.email.allowUnverifiedLogin` field** from your `main.wasp` file.
In Wasp 0.12.X we removed the `auth.methods.email.allowUnverifiedLogin` field to make our Email auth implementation easier to reason about. If you were using it, you should remove it from your `main.wasp` file.
3. Ensure your **local development database is running**.
4. **Do the schema migration** (create the new auth tables in the database) by running:
You should see the new `Auth`, `AuthIdentity` and `Session` tables in your database. You can use the `wasp db studio` command to open the database in a GUI and verify the tables are there. At the moment, they will be empty.
5. **Do the data migration** (move existing users from the old auth system to the new one by filling the new auth tables in the database with their data):
1. **Implement your data migration function(s)** in e.g. `src/migrateToNewAuth.ts`.
Below we prepared [examples of migration functions](#example-data-migration-functions) for each of the auth methods, for you to use as a starting point. They should be fine to use as-is, meaning you can just copy them and they are likely to work out of the box for typical use cases, but you can also modify them for your needs.
We recommend you create one function per each auth method that you use in your app.
2. **Define custom API endpoints for each migration function** you implemented.
With each data migration function below, we provided a relevant `api` declaration that you should add to your `main.wasp` file.
3. **Run the data migration function(s)** on the local development database by calling the API endpoints you defined in the previous step.
You can call the endpoint by visiting the URL in your browser, or by using a tool like `curl` or Postman.
For example, if you defined the API endpoint at `/migrate-username-and-password`, you can call it by visiting `http://localhost:3001/migrate-username-and-password` in your browser.
This should be it, you can now run `wasp db studio` again and verify that there is now relevant data in the new auth tables (`Auth` and `AuthIdentity`; `Session` should still be empty for now).
6. **Verify that the basic auth functionality works** by running `wasp start` and successfully signing up / logging in with each of the auth methods.
7. **Update your JS/TS code** to work correctly with the new auth.
You might want to use the new auth helper functions to get the `email` or `username` from a user object. For example, `user.username` might not work anymore for you, since the `username` obtained by the Username & Password auth method isn't stored on the `User` entity anymore (unless you are explicitly storing something into `user.username`, e.g. via `userSignupFields` for a social auth method like Github). Same goes for `email` from Email auth method.
Instead, you can now use `getUsername(user)` to get the username obtained from Username & Password auth method, or `getEmail(user)` to get the email obtained from Email auth method.
Read more about the helpers in the [Auth Entities - Accessing the Auth Fields](https://wasp-lang.dev/docs/auth/entities#accessing-the-auth-fields) section.
8. Finally, **check that your app now fully works as it worked before**. If all the above steps were done correctly, everything should be working now.
Migrating a deployed app
After successfully performing migration locally so far, and verifying that your app works as expected, it is time to also migrate our deployed app.
Before migrating your production (deployed) app, we advise you to back up your production database in case something goes wrong. Also, besides testing it in development, it's good to test the migration in a staging environment if you have one.
We will perform the production migration in 2 steps:
* Deploying the new code to production (client and server).
* Migrating the production database data.
* * *
Between these two steps, so after successfully deploying the new code to production and before migrating the production database data, your app will not be working completely: new users will be able to sign up, but existing users won't be able to log in, and already logged in users will be logged out. Once you do the second step, migrating the production database data, it will all be back to normal. You will likely want to keep the time between the two steps as short as you can.
* * *
* **First step: deploy the new code** (client and server), either via `wasp deploy` (i.e. `wasp deploy fly deploy`) or manually.
Check our [Deployment docs](https://wasp-lang.dev/docs/advanced/deployment/overview) for more details.
* **Second step: run the data migration functions** on the production database.
You can do this by calling the API endpoints you defined in the previous step, just like you did locally. You can call the endpoint by visiting the URL in your browser, or by using a tool like `curl` or Postman.
For example, if you defined the API endpoint at `/migrate-username-and-password`, you can call it by visiting `https://your-server-url.com/migrate-username-and-password` in your browser.
Your deployed app should be working normally now, with the new auth system.
#### 2\. Cleanup the Old Auth System[](#2-cleanup-the-old-auth-system "Direct link to 2. Cleanup the Old Auth System")
Your app should be working correctly and using new auth, but to finish the migration, we need to clean up the old auth system:
1. In `main.wasp` file, **delete auth-related fields from the `User` entity**, since with 0.12 they got moved to the internal Wasp entity `AuthIdentity`.
* This means any fields that were required by Wasp for authentication, like `email`, `password`, `isEmailVerified`, `emailVerificationSentAt`, `passwordResetSentAt`, `username`, etc.
* There are situations in which you might want to keep some of them, e.g. `email` and/or `username`, if they are still relevant for you due to your custom logic (e.g. you are populating them with `userSignupFields` upon social signup in order to have this info easily available on the `User` entity). Note that they won't be used by Wasp Auth anymore, they are here just for your business logic.
2. In `main.wasp` file, **remove the `externalAuthEntity` field from the `app.auth`** and also **remove the whole `SocialLogin` entity** if you used Google or GitHub auth.
3. **Delete the data migration function(s)** you implemented earlier (e.g. in `src/migrateToNewAuth.ts`) and also the corresponding API endpoints from the `main.wasp` file.
4. **Run `wasp db migrate-dev`** again to apply these changes and remove the redundant fields from the database.
Migrating a deployed app
After doing the steps above successfully locally and making sure everything is working, it is time to push these changes to the deployed app again.
_Deploy the app again_, either via `wasp deploy` or manually. Check our [Deployment docs](https://wasp-lang.dev/docs/advanced/deployment/overview) for more details.
The database migrations will automatically run on successful deployment of the server and delete the now redundant auth-related `User` columns from the database.
Your app is now fully migrated to the new auth system.
### Next Steps[](#next-steps "Direct link to Next Steps")
If you made it this far, you've completed all the necessary steps to get your Wasp app working with Wasp 0.12.x. Nice work!
Finally, since Wasp no longer requires you to separate your client source files (previously in `src/client`) from server source files (previously in `src/server`), you are now free to reorganize your project however you think is best, as long as you keep all the source files in the `src/` directory.
This section is optional, but if you didn't like the server/client separation, now's the perfect time to change it.
For example, if your `src` dir looked like this:
```
src│├── client│ ├── Dashboard.tsx│ ├── Login.tsx│ ├── MainPage.tsx│ ├── Register.tsx│ ├── Task.css│ ├── TaskLisk.tsx│ ├── Task.tsx│ └── User.tsx├── server│ ├── taskActions.ts│ ├── taskQueries.ts│ ├── userActions.ts│ └── userQueries.ts└── shared └── utils.ts
```
you can now change it to a feature-based structure (which we recommend for any project that is not very small):
```
src│├── task│ ├── actions.ts -- former taskActions.ts│ ├── queries.ts -- former taskQueries.ts│ ├── Task.css│ ├── TaskLisk.tsx│ └── Task.tsx├── user│ ├── actions.ts -- former userActions.ts│ ├── Dashboard.tsx│ ├── Login.tsx│ ├── queries.ts -- former userQueries.ts│ ├── Register.tsx│ └── User.tsx├── MainPage.tsx└── utils.ts
```
## Appendix[](#appendix "Direct link to Appendix")
### Example Data Migration Functions[](#example-data-migration-functions "Direct link to Example Data Migration Functions")
The migration functions provided below are written with the typical use cases in mind and you can use them as-is. If your setup requires additional logic, you can use them as a good starting point and modify them to your needs.
Note that all of the functions below are written to be idempotent, meaning that running a function multiple times can't hurt. This allows executing a function again in case only a part of the previous execution succeeded and also means that accidentally running it one time too much won't have any negative effects. **We recommend you keep your data migration functions idempotent**.
#### Username & Password[](#username--password "Direct link to Username & Password")
To successfully migrate the users using the Username & Password auth method, you will need to do two things:
1. Migrate the user data
Username & Password data migration function
2. Provide a way for users to migrate their password
There is a **breaking change between the old and the new auth in the way the password is hashed**. This means that users will need to migrate their password after the migration, as the old password will no longer work.
Since the only way users using username and password as a login method can verify their identity is by providing both their username and password (there is no email or any other info, unless you asked for it and stored it explicitly), we need to provide them a way to **exchange their old password for a new password**. One way to handle this is to inform them about the need to migrate their password (on the login page) and provide a custom page to migrate the password.
Steps to create a custom page for migrating the password
#### Email[](#email "Direct link to Email")
To successfully migrate the users using the Email auth method, you will need to do two things:
1. Migrate the user data
Email data migration function
2. Ask the users to reset their password
There is a **breaking change between the old and the new auth in the way the password is hashed**. This means that users will need to reset their password after the migration, as the old password will no longer work.
It would be best to notify your users about this change and put a notice on your login page to **request a password reset**.
#### Google & GitHub[](#google--github "Direct link to Google & GitHub")
Google & GitHub data migration functions | null | https://wasp-lang.dev/docs/migrate-from-0-11-to-0-12 | To fully migrate from 0.11.X to the latest version of Wasp, you should first migrate to 0.12.X and then to 0.13.X. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ec9756afb711c-YYZ | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:11:35 GMT | Tue, 23 Apr 2024 15:21:35 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=OlgO33mJlbn92QyVkI%2Bd1bo3ZUxkBuDZcIHRkvFNYLQWWf2190Vs8%2BD0leT7n3a4RpnE%2BC266W%2FG3RnM7%2BpO1gkOOJehTCfSIf6ysvZhUmxqQFi2AJoyPy6tNEdfYRkt"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | e6ffbd438a4d166142b616c9f6ee87c51c849830 | h2 | 6876:FF8F1:395BE21:443AC8E:6627CFA6 | HIT | MISS | cache-yyz4551-YYZ | S1713885095.284924, VS0, VE36 | null | null | en | og:image | https://wasp-lang.dev/docs/migrate-from-0-11-to-0-12 | og:url | Migration from 0.11.X to 0.12.X | Wasp | og:title | To fully migrate from 0.11.X to the latest version of Wasp, you should first migrate to 0.12.X and then to 0.13.X. | og:description | Migration from 0.11.X to 0.12.X | Wasp | null | The latest version of Wasp is 0.13.X
To fully migrate from 0.11.X to the latest version of Wasp, you should first migrate to 0.12.X and then to 0.13.X.
Make sure to read the migration guide from 0.12.X to 0.13.X after you finish this one.
What's new in Wasp 0.12.0?
New project structure
Here's a file tree of a fresh Wasp project created with the previous version of Wasp. More precisely, this is what you'll get if you run wasp new myProject using Wasp 0.11.x:
.
├── .gitignore
├── main.wasp
├── src
│ ├── client
│ │ ├── Main.css
│ │ ├── MainPage.jsx
│ │ ├── react-app-env.d.ts
│ │ ├── tsconfig.json
│ │ └── waspLogo.png
│ ├── server
│ │ └── tsconfig.json
│ ├── shared
│ │ └── tsconfig.json
│ └── .waspignore
└── .wasproot
Compare that with the file tree of a fresh Wasp project created with Wasp 0.12.0. In other words, this is what you will get by running wasp new myProject from this point onwards:
.
├── .gitignore
├── main.wasp
├── package.json
├── public
│ └── .gitkeep
├── src
│ ├── Main.css
│ ├── MainPage.jsx
│ ├── queries.ts
│ ├── vite-env.d.ts
│ ├── .waspignore
│ └── waspLogo.png
├── tsconfig.json
├── vite.config.ts
└── .wasproot
The main differences are:
The server/client code separation is no longer necessary. You can now organize your code however you want, as long as it's inside the src directory.
All external imports in your Wasp file must have paths starting with @src (e.g., import foo from '@src/bar.js') where @src refers to the src directory in your project root. The paths can no longer start with @server or @client.
Your project now features a top-level public dir. Wasp will publicly serve all the files it finds in this directory. Read more about it here.
Our Overview docs explain the new structure in detail, while this page provides a quick guide for migrating existing projects.
New auth
In Wasp 0.11.X, authentication was based on the User model which the developer needed to set up properly and take care of the auth fields like email or password.
main.wasp
app myApp {
wasp: {
version: "^0.11.0"
},
title: "My App",
auth: {
userEntity: User,
externalAuthEntity: SocialLogin,
methods: {
gitHub: {}
},
onAuthFailedRedirectTo: "/login"
},
}
entity User {=psl
id Int @id @default(autoincrement())
username String @unique
password String
externalAuthAssociations SocialLogin[]
psl=}
entity SocialLogin {=psl
id Int @id @default(autoincrement())
provider String
providerId String
user User @relation(fields: [userId], references: [id], onDelete: Cascade)
userId Int
createdAt DateTime @default(now())
@@unique([provider, providerId, userId])
psl=}
From 0.12.X onwards, authentication is based on the auth models which are automatically set up by Wasp. You don't need to take care of the auth fields anymore.
The User model is now just a business logic model and you use it for storing the data that is relevant for your app.
main.wasp
app myApp {
wasp: {
version: "^0.12.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
gitHub: {}
},
onAuthFailedRedirectTo: "/login"
},
}
entity User {=psl
id Int @id @default(autoincrement())
psl=}
Regression Note: Multiple Auth Identities per User
With our old auth implementation, if you were using both Google and email auth methods, your users could sign up with Google first and then, later on, reset their password and therefore also enable logging in with their email and password. This was the only way in which a single user could have multiple login methods at the same time (Google and email).
This is not possible anymore. The new auth system doesn't support multiple login methods per user at the moment. We do plan to add this soon though, with the introduction of the account merging feature.
If you have any users that have both Google and email login credentials at the same time, you will have to pick only one of those for that user to keep when migrating them.
Regression Note: _waspCustomValidations is deprecated
Auth field customization is no longer possible using the _waspCustomValidations on the User entity. This is a part of auth refactoring that we are doing to make it easier to customize auth. We will be adding more customization options in the future.
You can read more about the new auth system in the Auth Entities section.
How to Migrate?
These instructions are for migrating your app from Wasp 0.11.X to Wasp 0.12.X, meaning they will work for all minor releases that fit this pattern (e.g., the guide applies to 0.12.0, 0.12.1, ...).
The guide consists of two big steps:
Migrating your Wasp project to the new structure.
Migrating to the new auth.
If you get stuck at any point, don't hesitate to ask for help on our Discord server.
Migrating Your Project to the New Structure
You can easily migrate your old Wasp project to the new structure by following a series of steps. Assuming you have a project called foo inside the directory foo, you should:
Install the 0.12.x version of Wasp.
curl -sSL https://get.wasp-lang.dev/installer.sh | sh -s -- -v 0.12.4
Make sure to backup or save your project before starting the procedure (e.g., by committing it to source control or creating a copy).
Position yourself in the terminal in the directory that is a parent of your wasp project directory (so one level above: if you do ls, you should see your wasp project dir listed).
Run the migration script (replace foo at the end with the name of your Wasp project directory) and follow the instructions:
In case the migration script doesn't work well for you, you can do the same steps manually, as described here:
That's it! You now have a properly structured Wasp 0.12.0 project in the foo directory. Your app probably doesn't quite work yet due to some other changes in Wasp 0.12.0, but we'll get to that in the next sections.
Migrating declaration names
Wasp 0.12.0 adds a casing constraints when naming Queries, Actions, Jobs, and Entities in the main.wasp file.
The following casing conventions have now become mandatory:
Operation (i.e., Query and Action) names must begin with a lowercase letter: query getTasks {...}, action createTask {...}.
Job names must begin with a lowercase letter: job sendReport {...}.
Entity names must start with an uppercase letter: entity Task {...}.
Migrating the Tailwind Setup
note
If you don't use Tailwind in your project, you can skip this section.
There is a small change in how the tailwind.config.cjs needs to be defined in Wasp 0.12.0.
You'll need to wrap all your paths in the content field with the resolveProjectPath function. This makes sure that the paths are resolved correctly when generating your CSS.
Here's how you can do it:
Before
After
tailwind.config.cjs
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
'./src/**/*.{js,jsx,ts,tsx}',
],
theme: {
extend: {},
},
plugins: [],
}
Default Server Dockerfile Changed
note
If you didn't customize your Dockerfile or had a custom build process for the Wasp server, you can skip this section.
Between Wasp 0.11.X and 0.12.X, the Dockerfile that Wasp generates for you for deploying the server has changed. If you defined a custom Dockerfile in your project root dir or in any other way relied on its contents, you'll need to update it to incorporate the changes that Wasp 0.12.X made.
We suggest that you temporarily move your custom Dockerfile to a different location, then run wasp start to generate the new Dockerfile. Check out the .wasp/out/Dockerfile to see the new Dockerfile and what changes you need to make. You'll probably need to copy some of the changes from the new Dockerfile to your custom one to make your app work with Wasp 0.12.X.
Migrating to the New Auth
As shown in the previous section, Wasp significantly changed how authentication works in version 0.12.0. This section leads you through migrating your app from Wasp 0.11.X to Wasp 0.12.X.
Migrating your existing app to the new auth system is a two-step process:
Migrate to the new auth system
Clean up the old auth system
Migrating a deployed app
While going through these steps, we will focus first on doing the changes locally (including your local development database).
Once we confirm everything works well locally, we will apply the same changes to the deployed app (including your production database).
We'll put extra info for migrating a deployed app in a box like this one.
1. Migrate to the New Auth System
You can follow these steps to migrate to the new auth system (assuming you already migrated the project structure to 0.12, as described above):
Migrate getUserFields and/or additionalSignupFields in the main.wasp file to the new userSignupFields field.
If you are not using them, you can skip this step.
In Wasp 0.11.X, you could define a getUserFieldsFn to specify extra fields that would get saved to the User when using Google or GitHub to sign up.
You could also define additionalSignupFields to specify extra fields for the Email or Username & Password signup.
In 0.12.X, we unified these two concepts into the userSignupFields field.
Migration for Email and Username & PasswordMigration for Github and Google
Remove the auth.methods.email.allowUnverifiedLogin field from your main.wasp file.
In Wasp 0.12.X we removed the auth.methods.email.allowUnverifiedLogin field to make our Email auth implementation easier to reason about. If you were using it, you should remove it from your main.wasp file.
Ensure your local development database is running.
Do the schema migration (create the new auth tables in the database) by running:
You should see the new Auth, AuthIdentity and Session tables in your database. You can use the wasp db studio command to open the database in a GUI and verify the tables are there. At the moment, they will be empty.
Do the data migration (move existing users from the old auth system to the new one by filling the new auth tables in the database with their data):
Implement your data migration function(s) in e.g. src/migrateToNewAuth.ts.
Below we prepared examples of migration functions for each of the auth methods, for you to use as a starting point. They should be fine to use as-is, meaning you can just copy them and they are likely to work out of the box for typical use cases, but you can also modify them for your needs.
We recommend you create one function per each auth method that you use in your app.
Define custom API endpoints for each migration function you implemented.
With each data migration function below, we provided a relevant api declaration that you should add to your main.wasp file.
Run the data migration function(s) on the local development database by calling the API endpoints you defined in the previous step.
You can call the endpoint by visiting the URL in your browser, or by using a tool like curl or Postman.
For example, if you defined the API endpoint at /migrate-username-and-password, you can call it by visiting http://localhost:3001/migrate-username-and-password in your browser.
This should be it, you can now run wasp db studio again and verify that there is now relevant data in the new auth tables (Auth and AuthIdentity; Session should still be empty for now).
Verify that the basic auth functionality works by running wasp start and successfully signing up / logging in with each of the auth methods.
Update your JS/TS code to work correctly with the new auth.
You might want to use the new auth helper functions to get the email or username from a user object. For example, user.username might not work anymore for you, since the username obtained by the Username & Password auth method isn't stored on the User entity anymore (unless you are explicitly storing something into user.username, e.g. via userSignupFields for a social auth method like Github). Same goes for email from Email auth method.
Instead, you can now use getUsername(user) to get the username obtained from Username & Password auth method, or getEmail(user) to get the email obtained from Email auth method.
Read more about the helpers in the Auth Entities - Accessing the Auth Fields section.
Finally, check that your app now fully works as it worked before. If all the above steps were done correctly, everything should be working now.
Migrating a deployed app
After successfully performing migration locally so far, and verifying that your app works as expected, it is time to also migrate our deployed app.
Before migrating your production (deployed) app, we advise you to back up your production database in case something goes wrong. Also, besides testing it in development, it's good to test the migration in a staging environment if you have one.
We will perform the production migration in 2 steps:
Deploying the new code to production (client and server).
Migrating the production database data.
Between these two steps, so after successfully deploying the new code to production and before migrating the production database data, your app will not be working completely: new users will be able to sign up, but existing users won't be able to log in, and already logged in users will be logged out. Once you do the second step, migrating the production database data, it will all be back to normal. You will likely want to keep the time between the two steps as short as you can.
First step: deploy the new code (client and server), either via wasp deploy (i.e. wasp deploy fly deploy) or manually.
Check our Deployment docs for more details.
Second step: run the data migration functions on the production database.
You can do this by calling the API endpoints you defined in the previous step, just like you did locally. You can call the endpoint by visiting the URL in your browser, or by using a tool like curl or Postman.
For example, if you defined the API endpoint at /migrate-username-and-password, you can call it by visiting https://your-server-url.com/migrate-username-and-password in your browser.
Your deployed app should be working normally now, with the new auth system.
2. Cleanup the Old Auth System
Your app should be working correctly and using new auth, but to finish the migration, we need to clean up the old auth system:
In main.wasp file, delete auth-related fields from the User entity, since with 0.12 they got moved to the internal Wasp entity AuthIdentity.
This means any fields that were required by Wasp for authentication, like email, password, isEmailVerified, emailVerificationSentAt, passwordResetSentAt, username, etc.
There are situations in which you might want to keep some of them, e.g. email and/or username, if they are still relevant for you due to your custom logic (e.g. you are populating them with userSignupFields upon social signup in order to have this info easily available on the User entity). Note that they won't be used by Wasp Auth anymore, they are here just for your business logic.
In main.wasp file, remove the externalAuthEntity field from the app.auth and also remove the whole SocialLogin entity if you used Google or GitHub auth.
Delete the data migration function(s) you implemented earlier (e.g. in src/migrateToNewAuth.ts) and also the corresponding API endpoints from the main.wasp file.
Run wasp db migrate-dev again to apply these changes and remove the redundant fields from the database.
Migrating a deployed app
After doing the steps above successfully locally and making sure everything is working, it is time to push these changes to the deployed app again.
Deploy the app again, either via wasp deploy or manually. Check our Deployment docs for more details.
The database migrations will automatically run on successful deployment of the server and delete the now redundant auth-related User columns from the database.
Your app is now fully migrated to the new auth system.
Next Steps
If you made it this far, you've completed all the necessary steps to get your Wasp app working with Wasp 0.12.x. Nice work!
Finally, since Wasp no longer requires you to separate your client source files (previously in src/client) from server source files (previously in src/server), you are now free to reorganize your project however you think is best, as long as you keep all the source files in the src/ directory.
This section is optional, but if you didn't like the server/client separation, now's the perfect time to change it.
For example, if your src dir looked like this:
src
│
├── client
│ ├── Dashboard.tsx
│ ├── Login.tsx
│ ├── MainPage.tsx
│ ├── Register.tsx
│ ├── Task.css
│ ├── TaskLisk.tsx
│ ├── Task.tsx
│ └── User.tsx
├── server
│ ├── taskActions.ts
│ ├── taskQueries.ts
│ ├── userActions.ts
│ └── userQueries.ts
└── shared
└── utils.ts
you can now change it to a feature-based structure (which we recommend for any project that is not very small):
src
│
├── task
│ ├── actions.ts -- former taskActions.ts
│ ├── queries.ts -- former taskQueries.ts
│ ├── Task.css
│ ├── TaskLisk.tsx
│ └── Task.tsx
├── user
│ ├── actions.ts -- former userActions.ts
│ ├── Dashboard.tsx
│ ├── Login.tsx
│ ├── queries.ts -- former userQueries.ts
│ ├── Register.tsx
│ └── User.tsx
├── MainPage.tsx
└── utils.ts
Appendix
Example Data Migration Functions
The migration functions provided below are written with the typical use cases in mind and you can use them as-is. If your setup requires additional logic, you can use them as a good starting point and modify them to your needs.
Note that all of the functions below are written to be idempotent, meaning that running a function multiple times can't hurt. This allows executing a function again in case only a part of the previous execution succeeded and also means that accidentally running it one time too much won't have any negative effects. We recommend you keep your data migration functions idempotent.
Username & Password
To successfully migrate the users using the Username & Password auth method, you will need to do two things:
Migrate the user data
Username & Password data migration function
Provide a way for users to migrate their password
There is a breaking change between the old and the new auth in the way the password is hashed. This means that users will need to migrate their password after the migration, as the old password will no longer work.
Since the only way users using username and password as a login method can verify their identity is by providing both their username and password (there is no email or any other info, unless you asked for it and stored it explicitly), we need to provide them a way to exchange their old password for a new password. One way to handle this is to inform them about the need to migrate their password (on the login page) and provide a custom page to migrate the password.
Steps to create a custom page for migrating the password
Email
To successfully migrate the users using the Email auth method, you will need to do two things:
Migrate the user data
Email data migration function
Ask the users to reset their password
There is a breaking change between the old and the new auth in the way the password is hashed. This means that users will need to reset their password after the migration, as the old password will no longer work.
It would be best to notify your users about this change and put a notice on your login page to request a password reset.
Google & GitHub
Google & GitHub data migration functions | https://wasp-lang.dev/docs/migrate-from-0-11-to-0-12 |
|
1 | 200 | 2024-04-23T15:11:50.465Z | https://wasp-lang.dev/docs/migrate-from-0-12-to-0-13 | https://wasp-lang.dev/docs | browser | Are you on 0.11.X or earlier?
This guide only covers the migration from **0.12.X to 0.13.X**. If you are migrating from 0.11.X or earlier, please read the [migration guide from 0.11.X to 0.12.X](https://wasp-lang.dev/docs/migrate-from-0-11-to-0-12) first.
## What's new in 0.13.0?[](#whats-new-in-0130 "Direct link to What's new in 0.13.0?")
### OAuth providers got an overhaul[](#oauth-providers-got-an-overhaul "Direct link to OAuth providers got an overhaul")
Wasp 0.13.0 switches away from using Passport for our OAuth providers in favor of [Arctic](https://arctic.js.org/) from the [Lucia](https://lucia-auth.com/) ecosystem. This change simplifies the codebase and makes it easier to add new OAuth providers in the future.
### We added Keycloak as an OAuth provider[](#we-added-keycloak-as-an-oauth-provider "Direct link to We added Keycloak as an OAuth provider")
Wasp now supports using [Keycloak](https://www.keycloak.org/) as an OAuth provider.
## How to migrate?[](#how-to-migrate "Direct link to How to migrate?")
### Migrate your OAuth setup[](#migrate-your-oauth-setup "Direct link to Migrate your OAuth setup")
We had to make some breaking changes to upgrade the OAuth setup to the new Arctic lib.
Follow the steps below to migrate:
1. **Define the `WASP_SERVER_URL` server env variable**
In 0.13.0 Wasp introduces a new server env variable `WASP_SERVER_URL` that you need to define. This is the URL of your Wasp server and it's used to generate the redirect URL for the OAuth providers.
Server env variables
```
WASP_SERVER_URL=https://your-wasp-server-url.com
```
In development, Wasp sets the `WASP_SERVER_URL` to `http://localhost:3001` by default.
Migrating a deployed app
If you are migrating a deployed app, you will need to define the `WASP_SERVER_URL` server env variable in your deployment environment.
Read more about setting env variables in production [here](https://wasp-lang.dev/docs/project/env-vars#defining-env-vars-in-production).
2. **Update the redirect URLs** for the OAuth providers
The redirect URL for the OAuth providers has changed. You will need to update the redirect URL for the OAuth providers in the provider's dashboard.
* Before
* After
```
{clientUrl}/auth/login/{provider}
```
Check the new redirect URLs for [Google](https://wasp-lang.dev/docs/auth/social-auth/google#3-creating-a-google-oauth-app) and [GitHub](https://wasp-lang.dev/docs/auth/social-auth/github#3-creating-a-github-oauth-app) in Wasp's docs.
3. **Update the `configFn`** for the OAuth providers
If you didn't use the `configFn` option, you can skip this step.
If you used the `configFn` to configure the `scope` for the OAuth providers, you will need to rename the `scope` property to `scopes`.
Also, the object returned from `configFn` no longer needs to include the Client ID and the Client Secret. You can remove them from the object that `configFn` returns.
* Before
* After
google.ts
```
export function getConfig() { return { clientID: process.env.GOOGLE_CLIENT_ID, clientSecret: process.env.GOOGLE_CLIENT_SECRET, scope: ['profile', 'email'], }}
```
4. **Update the `userSignupFields` fields** to use the new `profile` format
If you didn't use the `userSignupFields` option, you can skip this step.
The data format for the `profile` that you receive from the OAuth providers has changed. You will need to update your code to reflect this change.
* Before
* After
google.ts
```
import { defineUserSignupFields } from 'wasp/server/auth'export const userSignupFields = defineUserSignupFields({ displayName: (data: any) => data.profile.displayName,})
```
Wasp now directly forwards what it receives from the OAuth providers. You can check the data format for [Google](https://wasp-lang.dev/docs/auth/social-auth/google#data-received-from-google) and [GitHub](https://wasp-lang.dev/docs/auth/social-auth/github#data-received-from-github) in Wasp's docs.
That's it!
You should now be able to run your app with the new Wasp 0.13.0. | null | https://wasp-lang.dev/docs/migrate-from-0-12-to-0-13 | This guide only covers the migration from 0.12.X to 0.13.X. If you are migrating from 0.11.X or earlier, please read the migration guide from 0.11.X to 0.12.X first. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ec9a88a9b4bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:11:43 GMT | Tue, 23 Apr 2024 15:21:43 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=T7KPfv89Z9BAKDeE4ilSYgakTQTXUyLGuLWahZ2efc2IH6qnW9%2FPKGUyo4dTAnPhWHiuH%2F1%2B14an5%2BVO5D98OED8%2Fs9hv%2Bm11WK0IkN39ZWMr4VUR8eN%2B0Ap2DKOIHmO"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | f9ac6a7c9b201d98a22f73a447242ba70c03281d | h2 | 5A92:180046:3C24A37:47297FB:6627CFAF | null | MISS | cache-nyc-kteb1890087-NYC | S1713885103.452461, VS0, VE24 | null | null | en | og:image | https://wasp-lang.dev/docs/migrate-from-0-12-to-0-13 | og:url | Migration from 0.12.X to 0.13.X | Wasp | og:title | This guide only covers the migration from 0.12.X to 0.13.X. If you are migrating from 0.11.X or earlier, please read the migration guide from 0.11.X to 0.12.X first. | og:description | Migration from 0.12.X to 0.13.X | Wasp | null | Are you on 0.11.X or earlier?
This guide only covers the migration from 0.12.X to 0.13.X. If you are migrating from 0.11.X or earlier, please read the migration guide from 0.11.X to 0.12.X first.
What's new in 0.13.0?
OAuth providers got an overhaul
Wasp 0.13.0 switches away from using Passport for our OAuth providers in favor of Arctic from the Lucia ecosystem. This change simplifies the codebase and makes it easier to add new OAuth providers in the future.
We added Keycloak as an OAuth provider
Wasp now supports using Keycloak as an OAuth provider.
How to migrate?
Migrate your OAuth setup
We had to make some breaking changes to upgrade the OAuth setup to the new Arctic lib.
Follow the steps below to migrate:
Define the WASP_SERVER_URL server env variable
In 0.13.0 Wasp introduces a new server env variable WASP_SERVER_URL that you need to define. This is the URL of your Wasp server and it's used to generate the redirect URL for the OAuth providers.
Server env variables
WASP_SERVER_URL=https://your-wasp-server-url.com
In development, Wasp sets the WASP_SERVER_URL to http://localhost:3001 by default.
Migrating a deployed app
If you are migrating a deployed app, you will need to define the WASP_SERVER_URL server env variable in your deployment environment.
Read more about setting env variables in production here.
Update the redirect URLs for the OAuth providers
The redirect URL for the OAuth providers has changed. You will need to update the redirect URL for the OAuth providers in the provider's dashboard.
Before
After
{clientUrl}/auth/login/{provider}
Check the new redirect URLs for Google and GitHub in Wasp's docs.
Update the configFn for the OAuth providers
If you didn't use the configFn option, you can skip this step.
If you used the configFn to configure the scope for the OAuth providers, you will need to rename the scope property to scopes.
Also, the object returned from configFn no longer needs to include the Client ID and the Client Secret. You can remove them from the object that configFn returns.
Before
After
google.ts
export function getConfig() {
return {
clientID: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
scope: ['profile', 'email'],
}
}
Update the userSignupFields fields to use the new profile format
If you didn't use the userSignupFields option, you can skip this step.
The data format for the profile that you receive from the OAuth providers has changed. You will need to update your code to reflect this change.
Before
After
google.ts
import { defineUserSignupFields } from 'wasp/server/auth'
export const userSignupFields = defineUserSignupFields({
displayName: (data: any) => data.profile.displayName,
})
Wasp now directly forwards what it receives from the OAuth providers. You can check the data format for Google and GitHub in Wasp's docs.
That's it!
You should now be able to run your app with the new Wasp 0.13.0. | https://wasp-lang.dev/docs/migrate-from-0-12-to-0-13 |
|
1 | 200 | 2024-04-23T15:11:58.965Z | https://wasp-lang.dev/docs/0.12.0 | https://wasp-lang.dev/docs | browser | ## Introduction
note
If you are looking for the installation instructions, check out the [Quick Start](https://wasp-lang.dev/docs/0.12.0/quick-start) section.
We will give a brief overview of what Wasp is, how it works on a high level and when to use it.
It is an opinionated way of building **full-stack web applications**. It takes care of all three major parts of a web application: **client** (front-end), **server** (back-end) and **database**.
### Works well with your existing stack[](#works-well-with-your-existing-stack "Direct link to Works well with your existing stack")
Wasp is not trying to do everything at once but rather focuses on the complexity which arises from connecting all the parts of the stack (client, server, database, deployment) together.
Wasp is using **React**, **Node.js** and **Prisma** under the hood and relies on them to define web components and server queries and actions.
### Wasp's secret sauce[](#wasps-secret-sauce "Direct link to Wasp's secret sauce")
At the core is the Wasp compiler which takes the Wasp config and your Javascript code and outputs the client app, server app and deployment code.
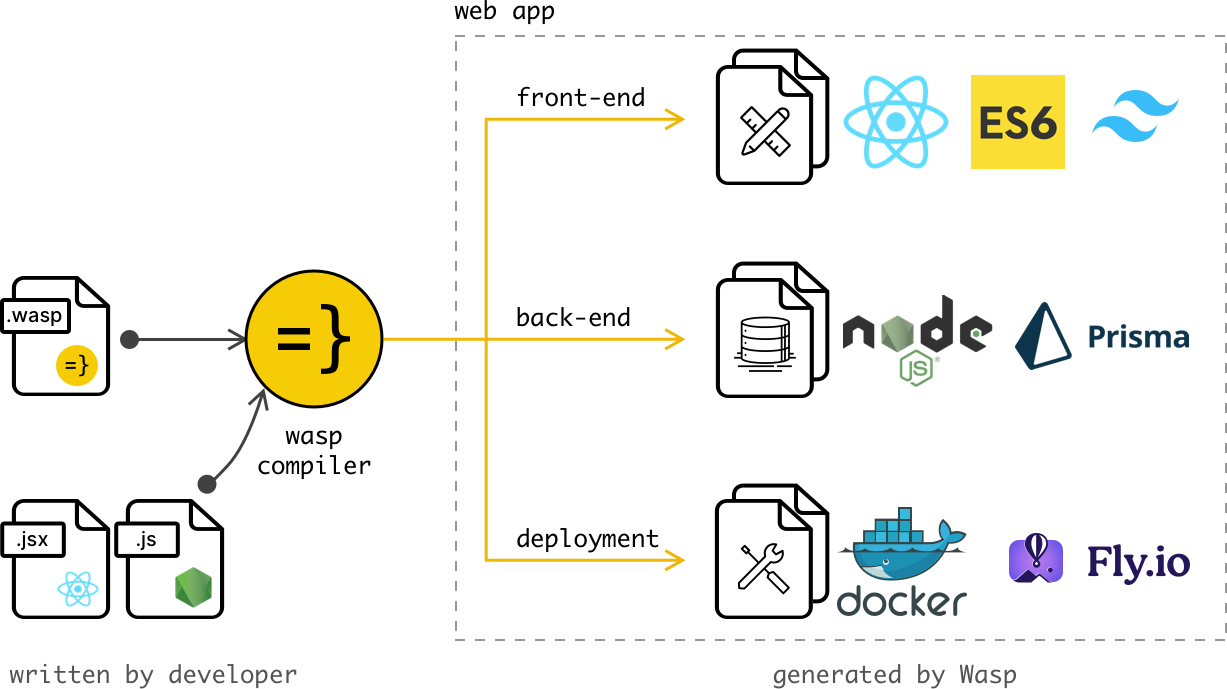
How the magic happens 🌈
The cool thing about having a compiler that understands your code is that it can do a lot of things for you.
Define your app in the Wasp config and get:
* login and signup with Auth UI components,
* full-stack type safety,
* e-mail sending,
* async processing jobs,
* React Query powered data fetching,
* security best practices,
* and more.
You don't need to write any code for these features, Wasp will take care of it for you 🤯 And what's even better, Wasp also maintains the code for you, so you don't have to worry about keeping up with the latest security best practices. As Wasp updates, so does your app.
## So what does the code look like?[](#so-what-does-the-code-look-like "Direct link to So what does the code look like?")
Let's say you want to build a web app that allows users to **create and share their favorite recipes**.
Let's start with the main.wasp file: it is the central file of your app, where you describe the app from the high level.
Let's give our app a title and let's immediately turn on the full-stack authentication via username and password:
main.wasp
```
app RecipeApp { title: "My Recipes", wasp: { version: "^0.12.0" }, auth: { methods: { usernameAndPassword: {} }, onAuthFailedRedirectTo: "/login", userEntity: User }}
```
Let's then add the data models for your recipes. We will want to have Users and Users can own Recipes:
main.wasp
```
...entity User {=psl // Data models are defined using Prisma Schema Language. id Int @id @default(autoincrement()) recipes Recipe[]psl=}entity Recipe {=psl id Int @id @default(autoincrement()) title String description String? userId Int user User @relation(fields: [userId], references: [id])psl=}
```
Next, let's define how to do something with these data models!
We do that by defining Operations, in this case a Query `getRecipes` and Action `addRecipe`, which are in their essence a Node.js functions that execute on server and can, thanks to Wasp, very easily be called from the client.
First, we define these Operations in our main.wasp file, so Wasp knows about them and can "beef them up":
main.wasp
```
// Queries have automatic cache invalidation and are type-safe.query getRecipes { fn: import { getRecipes } from "@src/recipe/operations.ts", entities: [Recipe],}// Actions are type-safe and can be used to perform side-effects.action addRecipe { fn: import { addRecipe } from "@src/recipe/operations.ts", entities: [Recipe],}
```
... and then implement them in our Javascript (or TypeScript) code (we show just the query here, using TypeScript):
src/recipe/operations.ts
```
// Wasp generates the types for you.import { type GetRecipes } from "wasp/server/operations";import { type Recipe } from "wasp/entities";export const getRecipes: GetRecipes<{}, Recipe[]> = async (_args, context) => { return context.entities.Recipe.findMany( // Prisma query { where: { user: { id: context.user.id } } } );};export const addRecipe ...
```
Now we can very easily use these in our React components!
For the end, let's create a home page of our app.
First we define it in main.wasp:
main.wasp
```
...route HomeRoute { path: "/", to: HomePage }page HomePage { component: import { HomePage } from "@src/pages/HomePage", authRequired: true // Will send user to /login if not authenticated.}
```
and then implement it as a React component in JS/TS (that calls the Operations we previously defined):
src/pages/HomePage.tsx
```
import { useQuery, getRecipes } from "wasp/client/operations";import { type User } from "wasp/entities";export function HomePage({ user }: { user: User }) { // Due to full-stack type safety, `recipes` will be of type `Recipe[]` here. const { data: recipes, isLoading } = useQuery(getRecipes); // Calling our query here! if (isLoading) { return <div>Loading...</div>; } return ( <div> <h1>Recipes</h1> <ul> {recipes ? recipes.map((recipe) => ( <li key={recipe.id}> <div>{recipe.title}</div> <div>{recipe.description}</div> </li> )) : 'No recipes defined yet!'} </ul> </div> );}
```
And voila! We are listing all the recipes in our app 🎉
This was just a quick example to give you a taste of what Wasp is. For step by step tour through the most important Wasp features, check out the [Todo app tutorial](https://wasp-lang.dev/docs/0.12.0/tutorial/create).
note
Above we skipped defining `/login` and `/signup` pages to keep the example a bit shorter, but those are very simple to do by using Wasp's Auth UI feature.
## When to use Wasp[](#when-to-use-wasp "Direct link to When to use Wasp")
Wasp is addressing the same core problems that typical web app frameworks are addressing, and it in big part [looks, swims and quacks](https://en.wikipedia.org/wiki/Duck_test) like a web app framework.
### Best used for[](#best-used-for "Direct link to Best used for")
* building full-stack web apps (like e.g. Airbnb or Asana)
* quickly starting a web app with industry best practices
* to be used alongside modern web dev stack (currently supported React and Node)
### Avoid using Wasp for[](#avoid-using-wasp-for "Direct link to Avoid using Wasp for")
* building static/presentational websites
* to be used as a no-code solution
* to be a solve-it-all tool in a single language
## Wasp is a DSL[](#wasp-is-a-dsl "Direct link to Wasp is a DSL")
note
You don't need to know what a DSL is to use Wasp, but if you are curious, you can read more about it below.
Wasp does not match typical expectations of a web app framework: it is not a set of libraries, it is instead a simple programming language that understands your code and can do a lot of things for you.
Wasp is a programming language, but a specific kind: it is specialized for a single purpose: **building modern web applications**. We call such languages _DSL_s (Domain Specific Language).
Other examples of _DSL_s that are often used today are e.g. _SQL_ for databases and _HTML_ for web page layouts. The main advantage and reason why _DSL_s exist is that they need to do only one task (e.g. database queries) so they can do it well and provide the best possible experience for the developer.
The same idea stands behind Wasp - a language that will allow developers to **build modern web applications with 10x less code and less stack-specific knowledge**. | null | https://wasp-lang.dev/docs/0.12.0 | If you are looking for the installation instructions, check out the Quick Start section. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ec9fb18d34bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:11:56 GMT | Tue, 23 Apr 2024 15:21:56 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=ZmII97N%2F2588DcOzDv3r8D70KVEZ8lh8pUyE748uqW8qHloUL2S0d2Vrj6c3b8D6BCKce7NQUaoqy%2BZYa9GS5DNMnLXRfORnLa9rPU5Ie3OMFNAfOoIPgoBc9CgJbNcm"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 300a3546a810c9f34ed3e3853614d5732f7184e7 | h2 | 14AC:3F9EB3:3A3188F:4535AA4:6627CFBB | HIT | MISS | cache-nyc-kteb1890087-NYC | S1713885117.667298, VS0, VE16 | null | null | en | og:image | https://wasp-lang.dev/docs/0.12.0 | og:url | Introduction | Wasp | og:title | If you are looking for the installation instructions, check out the Quick Start section. | og:description | Introduction | Wasp | null | Introduction
note
If you are looking for the installation instructions, check out the Quick Start section.
We will give a brief overview of what Wasp is, how it works on a high level and when to use it.
It is an opinionated way of building full-stack web applications. It takes care of all three major parts of a web application: client (front-end), server (back-end) and database.
Works well with your existing stack
Wasp is not trying to do everything at once but rather focuses on the complexity which arises from connecting all the parts of the stack (client, server, database, deployment) together.
Wasp is using React, Node.js and Prisma under the hood and relies on them to define web components and server queries and actions.
Wasp's secret sauce
At the core is the Wasp compiler which takes the Wasp config and your Javascript code and outputs the client app, server app and deployment code.
How the magic happens 🌈
The cool thing about having a compiler that understands your code is that it can do a lot of things for you.
Define your app in the Wasp config and get:
login and signup with Auth UI components,
full-stack type safety,
e-mail sending,
async processing jobs,
React Query powered data fetching,
security best practices,
and more.
You don't need to write any code for these features, Wasp will take care of it for you 🤯 And what's even better, Wasp also maintains the code for you, so you don't have to worry about keeping up with the latest security best practices. As Wasp updates, so does your app.
So what does the code look like?
Let's say you want to build a web app that allows users to create and share their favorite recipes.
Let's start with the main.wasp file: it is the central file of your app, where you describe the app from the high level.
Let's give our app a title and let's immediately turn on the full-stack authentication via username and password:
main.wasp
app RecipeApp {
title: "My Recipes",
wasp: { version: "^0.12.0" },
auth: {
methods: { usernameAndPassword: {} },
onAuthFailedRedirectTo: "/login",
userEntity: User
}
}
Let's then add the data models for your recipes. We will want to have Users and Users can own Recipes:
main.wasp
...
entity User {=psl // Data models are defined using Prisma Schema Language.
id Int @id @default(autoincrement())
recipes Recipe[]
psl=}
entity Recipe {=psl
id Int @id @default(autoincrement())
title String
description String?
userId Int
user User @relation(fields: [userId], references: [id])
psl=}
Next, let's define how to do something with these data models!
We do that by defining Operations, in this case a Query getRecipes and Action addRecipe, which are in their essence a Node.js functions that execute on server and can, thanks to Wasp, very easily be called from the client.
First, we define these Operations in our main.wasp file, so Wasp knows about them and can "beef them up":
main.wasp
// Queries have automatic cache invalidation and are type-safe.
query getRecipes {
fn: import { getRecipes } from "@src/recipe/operations.ts",
entities: [Recipe],
}
// Actions are type-safe and can be used to perform side-effects.
action addRecipe {
fn: import { addRecipe } from "@src/recipe/operations.ts",
entities: [Recipe],
}
... and then implement them in our Javascript (or TypeScript) code (we show just the query here, using TypeScript):
src/recipe/operations.ts
// Wasp generates the types for you.
import { type GetRecipes } from "wasp/server/operations";
import { type Recipe } from "wasp/entities";
export const getRecipes: GetRecipes<{}, Recipe[]> = async (_args, context) => {
return context.entities.Recipe.findMany( // Prisma query
{ where: { user: { id: context.user.id } } }
);
};
export const addRecipe ...
Now we can very easily use these in our React components!
For the end, let's create a home page of our app.
First we define it in main.wasp:
main.wasp
...
route HomeRoute { path: "/", to: HomePage }
page HomePage {
component: import { HomePage } from "@src/pages/HomePage",
authRequired: true // Will send user to /login if not authenticated.
}
and then implement it as a React component in JS/TS (that calls the Operations we previously defined):
src/pages/HomePage.tsx
import { useQuery, getRecipes } from "wasp/client/operations";
import { type User } from "wasp/entities";
export function HomePage({ user }: { user: User }) {
// Due to full-stack type safety, `recipes` will be of type `Recipe[]` here.
const { data: recipes, isLoading } = useQuery(getRecipes); // Calling our query here!
if (isLoading) {
return <div>Loading...</div>;
}
return (
<div>
<h1>Recipes</h1>
<ul>
{recipes ? recipes.map((recipe) => (
<li key={recipe.id}>
<div>{recipe.title}</div>
<div>{recipe.description}</div>
</li>
)) : 'No recipes defined yet!'}
</ul>
</div>
);
}
And voila! We are listing all the recipes in our app 🎉
This was just a quick example to give you a taste of what Wasp is. For step by step tour through the most important Wasp features, check out the Todo app tutorial.
note
Above we skipped defining /login and /signup pages to keep the example a bit shorter, but those are very simple to do by using Wasp's Auth UI feature.
When to use Wasp
Wasp is addressing the same core problems that typical web app frameworks are addressing, and it in big part looks, swims and quacks like a web app framework.
Best used for
building full-stack web apps (like e.g. Airbnb or Asana)
quickly starting a web app with industry best practices
to be used alongside modern web dev stack (currently supported React and Node)
Avoid using Wasp for
building static/presentational websites
to be used as a no-code solution
to be a solve-it-all tool in a single language
Wasp is a DSL
note
You don't need to know what a DSL is to use Wasp, but if you are curious, you can read more about it below.
Wasp does not match typical expectations of a web app framework: it is not a set of libraries, it is instead a simple programming language that understands your code and can do a lot of things for you.
Wasp is a programming language, but a specific kind: it is specialized for a single purpose: building modern web applications. We call such languages DSLs (Domain Specific Language).
Other examples of DSLs that are often used today are e.g. SQL for databases and HTML for web page layouts. The main advantage and reason why DSLs exist is that they need to do only one task (e.g. database queries) so they can do it well and provide the best possible experience for the developer.
The same idea stands behind Wasp - a language that will allow developers to build modern web applications with 10x less code and less stack-specific knowledge. | https://wasp-lang.dev/docs/0.12.0 |
|
1 | 200 | 2024-04-23T15:12:23.531Z | https://wasp-lang.dev/docs/0.11.8 | https://wasp-lang.dev/docs | browser | ## Introduction
note
If you are looking for the installation instructions, check out the [Quick Start](https://wasp-lang.dev/docs/0.11.8/quick-start) section.
We will give a brief overview of what Wasp is, how it works on a high level and when to use it.
It is an opinionated way of building **full-stack web applications**. It takes care of all three major parts of a web application: **client** (front-end), **server** (back-end) and **database**.
### Works well with your existing stack[](#works-well-with-your-existing-stack "Direct link to Works well with your existing stack")
Wasp is not trying to do everything at once but rather focuses on the complexity which arises from connecting all the parts of the stack (client, server, database, deployment) together.
Wasp is using **React**, **Node.js** and **Prisma** under the hood and relies on them to define web components and server queries and actions.
### Wasp's secret sauce[](#wasps-secret-sauce "Direct link to Wasp's secret sauce")
At the core is the Wasp compiler which takes the Wasp config and your Javascript code and outputs the client app, server app and deployment code.
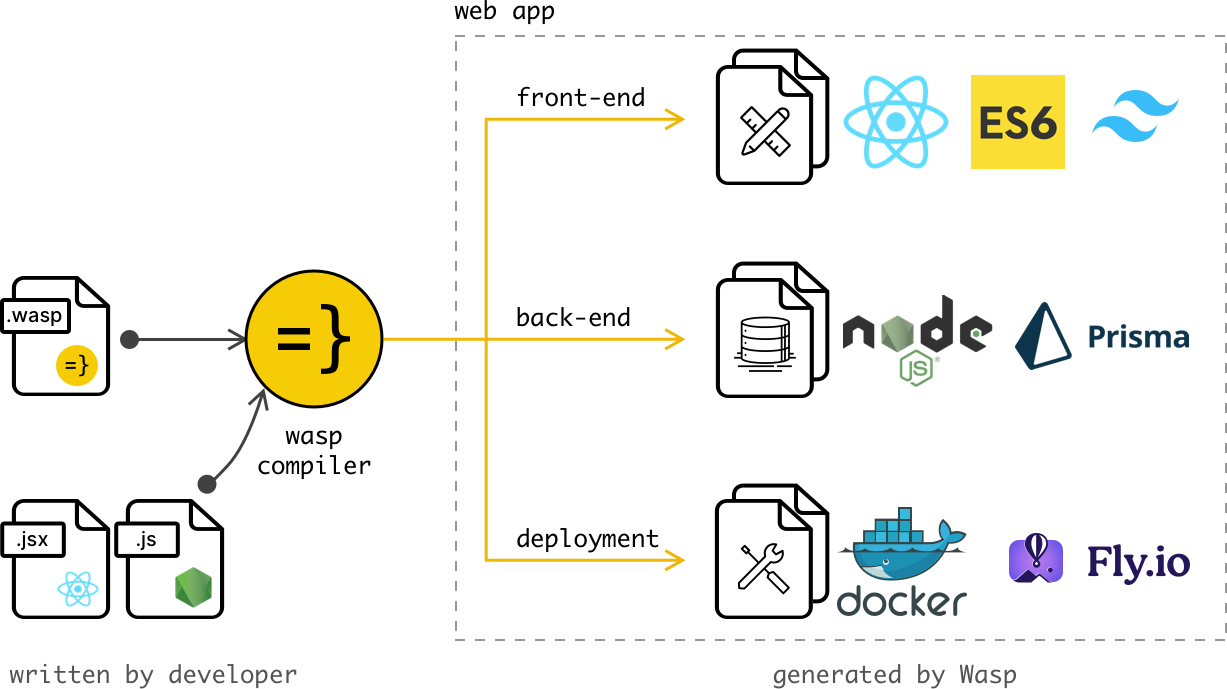
How the magic happens 🌈
The cool thing about having a compiler that understands your code is that it can do a lot of things for you.
Define your app in the Wasp config and get:
* login and signup with Auth UI components,
* full-stack type safety,
* e-mail sending,
* async processing jobs,
* React Query powered data fetching,
* security best practices,
* and more.
You don't need to write any code for these features, Wasp will take care of it for you 🤯 And what's even better, Wasp also maintains the code for you, so you don't have to worry about keeping up with the latest security best practices. As Wasp updates, so does your app.
## So what does the code look like?[](#so-what-does-the-code-look-like "Direct link to So what does the code look like?")
Let's say you want to build a web app that allows users to **create and share their favorite recipes**.
Let's start with the main.wasp file: it is the central file of your app, where you describe the app from the high level.
Let's give our app a title and let's immediately turn on the full-stack authentication via username and password:
main.wasp
```
app RecipeApp { title: "My Recipes", wasp: { version: "^0.11.0" }, auth: { methods: { usernameAndPassword: {} }, onAuthFailedRedirectTo: "/login", userEntity: User }}
```
Let's then add the data models for your recipes. We will want to have Users and Users can own Recipes:
main.wasp
```
...entity User {=psl // Data models are defined using Prisma Schema Language. id Int @id @default(autoincrement()) username String @unique password String recipes Recipe[]psl=}entity Recipe {=psl id Int @id @default(autoincrement()) title String description String? userId Int user User @relation(fields: [userId], references: [id])psl=}
```
Next, let's define how to do something with these data models!
We do that by defining Operations, in this case a Query `getRecipes` and Action `addRecipe`, which are in their essence a Node.js functions that execute on server and can, thanks to Wasp, very easily be called from the client.
First, we define these Operations in our main.wasp file, so Wasp knows about them and can "beef them up":
main.wasp
```
// Queries have automatic cache invalidation and are type-safe.query getRecipes { fn: import { getRecipes } from "@server/recipe.js", entities: [Recipe],}// Actions are type-safe and can be used to perform side-effects.action addRecipe { fn: import { addRecipe } from "@server/recipe.js", entities: [Recipe],}
```
... and then implement them in our Javascript (or TypeScript) code (we show just the query here, using TypeScript):
src/server/recipe.ts
```
// Wasp generates types for you.import type { GetRecipes } from "@wasp/queries/types";import type { Recipe } from "@wasp/entities";export const getRecipes: GetRecipes<{}, Recipe[]> = async (_args, context) => { return context.entities.Recipe.findMany( // Prisma query { where: { user: { id: context.user.id } } } );};export const addRecipe ...
```
Now we can very easily use these in our React components!
For the end, let's create a home page of our app.
First we define it in main.wasp:
main.wasp
```
...route HomeRoute { path: "/", to: HomePage }page HomePage { component: import { HomePage } from "@client/pages/HomePage", authRequired: true // Will send user to /login if not authenticated.}
```
and then implement it as a React component in JS/TS (that calls the Operations we previously defined):
src/client/pages/HomePage.tsx
```
import getRecipes from "@wasp/queries/getRecipes";import { useQuery } from "@wasp/queries";import type { User } from "@wasp/entities";export function HomePage({ user }: { user: User }) { // Due to full-stack type safety, `recipes` will be of type `Recipe[]` here. const { data: recipes, isLoading } = useQuery(getRecipes); // Calling our query here! if (isLoading) { return <div>Loading...</div>; } return ( <div> <h1>Recipes</h1> <ul> {recipes ? recipes.map((recipe) => ( <li key={recipe.id}> <div>{recipe.title}</div> <div>{recipe.description}</div> </li> )) : 'No recipes defined yet!'} </ul> </div> );}
```
And voila! We are listing all the recipes in our app 🎉
This was just a quick example to give you a taste of what Wasp is. For step by step tour through the most important Wasp features, check out the [Todo app tutorial](https://wasp-lang.dev/docs/0.11.8/tutorial/create).
note
Above we skipped defining /login and /signup pages to keep the example a bit shorter, but those are very simple to do by using Wasp's Auth UI feature.
## When to use Wasp[](#when-to-use-wasp "Direct link to When to use Wasp")
Wasp is addressing the same core problems that typical web app frameworks are addressing, and it in big part [looks, swims and quacks](https://en.wikipedia.org/wiki/Duck_test) like a web app framework.
### Best used for[](#best-used-for "Direct link to Best used for")
* building full-stack web apps (like e.g. Airbnb or Asana)
* quickly starting a web app with industry best practices
* to be used alongside modern web dev stack (currently supported React and Node)
### Avoid using Wasp for[](#avoid-using-wasp-for "Direct link to Avoid using Wasp for")
* building static/presentational websites
* to be used as a no-code solution
* to be a solve-it-all tool in a single language
## Wasp is a DSL[](#wasp-is-a-dsl "Direct link to Wasp is a DSL")
note
You don't need to know what a DSL is to use Wasp, but if you are curious, you can read more about it below.
Wasp does not match typical expectations of a web app framework: it is not a set of libraries, it is instead a programming language that understands your code and can do a lot of things for you.
Wasp is a programming language, but a specific kind: it is specialized for a single purpose: **building modern web applications**. We call such languages _DSL_s (Domain Specific Language).
Other examples of _DSL_s that are often used today are e.g. _SQL_ for databases and _HTML_ for web page layouts. The main advantage and reason why _DSL_s exist is that they need to do only one task (e.g. database queries) so they can do it well and provide the best possible experience for the developer.
The same idea stands behind Wasp - a language that will allow developers to **build modern web applications with 10x less code and less stack-specific knowledge**. | null | https://wasp-lang.dev/docs/0.11.8 | If you are looking for the installation instructions, check out the Quick Start section. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878eca945b6c4bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:12:21 GMT | Tue, 23 Apr 2024 15:22:21 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=I%2BWaqgUoqNTEH2rgf3Xh7Wgx5JpK%2BE3vTvc5TbN4hliC4MlM5AZB3wZNReuNmf3mE8scfpYncrfXwzztJXcWigmdMctVMLdcWdms%2Bn5BBgVHmlRCCBtDxrrUw4GDXYIO"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 57dc0972f9fc602cb2426a11f0b14c4d0ed9f4e9 | h2 | 51FC:173D:D2F35:1019FD:6627CFD5 | null | MISS | cache-nyc-kteb1890087-NYC | S1713885141.187041, VS0, VE19 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8 | og:url | Introduction | Wasp | og:title | If you are looking for the installation instructions, check out the Quick Start section. | og:description | Introduction | Wasp | null | Introduction
note
If you are looking for the installation instructions, check out the Quick Start section.
We will give a brief overview of what Wasp is, how it works on a high level and when to use it.
It is an opinionated way of building full-stack web applications. It takes care of all three major parts of a web application: client (front-end), server (back-end) and database.
Works well with your existing stack
Wasp is not trying to do everything at once but rather focuses on the complexity which arises from connecting all the parts of the stack (client, server, database, deployment) together.
Wasp is using React, Node.js and Prisma under the hood and relies on them to define web components and server queries and actions.
Wasp's secret sauce
At the core is the Wasp compiler which takes the Wasp config and your Javascript code and outputs the client app, server app and deployment code.
How the magic happens 🌈
The cool thing about having a compiler that understands your code is that it can do a lot of things for you.
Define your app in the Wasp config and get:
login and signup with Auth UI components,
full-stack type safety,
e-mail sending,
async processing jobs,
React Query powered data fetching,
security best practices,
and more.
You don't need to write any code for these features, Wasp will take care of it for you 🤯 And what's even better, Wasp also maintains the code for you, so you don't have to worry about keeping up with the latest security best practices. As Wasp updates, so does your app.
So what does the code look like?
Let's say you want to build a web app that allows users to create and share their favorite recipes.
Let's start with the main.wasp file: it is the central file of your app, where you describe the app from the high level.
Let's give our app a title and let's immediately turn on the full-stack authentication via username and password:
main.wasp
app RecipeApp {
title: "My Recipes",
wasp: { version: "^0.11.0" },
auth: {
methods: { usernameAndPassword: {} },
onAuthFailedRedirectTo: "/login",
userEntity: User
}
}
Let's then add the data models for your recipes. We will want to have Users and Users can own Recipes:
main.wasp
...
entity User {=psl // Data models are defined using Prisma Schema Language.
id Int @id @default(autoincrement())
username String @unique
password String
recipes Recipe[]
psl=}
entity Recipe {=psl
id Int @id @default(autoincrement())
title String
description String?
userId Int
user User @relation(fields: [userId], references: [id])
psl=}
Next, let's define how to do something with these data models!
We do that by defining Operations, in this case a Query getRecipes and Action addRecipe, which are in their essence a Node.js functions that execute on server and can, thanks to Wasp, very easily be called from the client.
First, we define these Operations in our main.wasp file, so Wasp knows about them and can "beef them up":
main.wasp
// Queries have automatic cache invalidation and are type-safe.
query getRecipes {
fn: import { getRecipes } from "@server/recipe.js",
entities: [Recipe],
}
// Actions are type-safe and can be used to perform side-effects.
action addRecipe {
fn: import { addRecipe } from "@server/recipe.js",
entities: [Recipe],
}
... and then implement them in our Javascript (or TypeScript) code (we show just the query here, using TypeScript):
src/server/recipe.ts
// Wasp generates types for you.
import type { GetRecipes } from "@wasp/queries/types";
import type { Recipe } from "@wasp/entities";
export const getRecipes: GetRecipes<{}, Recipe[]> = async (_args, context) => {
return context.entities.Recipe.findMany( // Prisma query
{ where: { user: { id: context.user.id } } }
);
};
export const addRecipe ...
Now we can very easily use these in our React components!
For the end, let's create a home page of our app.
First we define it in main.wasp:
main.wasp
...
route HomeRoute { path: "/", to: HomePage }
page HomePage {
component: import { HomePage } from "@client/pages/HomePage",
authRequired: true // Will send user to /login if not authenticated.
}
and then implement it as a React component in JS/TS (that calls the Operations we previously defined):
src/client/pages/HomePage.tsx
import getRecipes from "@wasp/queries/getRecipes";
import { useQuery } from "@wasp/queries";
import type { User } from "@wasp/entities";
export function HomePage({ user }: { user: User }) {
// Due to full-stack type safety, `recipes` will be of type `Recipe[]` here.
const { data: recipes, isLoading } = useQuery(getRecipes); // Calling our query here!
if (isLoading) {
return <div>Loading...</div>;
}
return (
<div>
<h1>Recipes</h1>
<ul>
{recipes ? recipes.map((recipe) => (
<li key={recipe.id}>
<div>{recipe.title}</div>
<div>{recipe.description}</div>
</li>
)) : 'No recipes defined yet!'}
</ul>
</div>
);
}
And voila! We are listing all the recipes in our app 🎉
This was just a quick example to give you a taste of what Wasp is. For step by step tour through the most important Wasp features, check out the Todo app tutorial.
note
Above we skipped defining /login and /signup pages to keep the example a bit shorter, but those are very simple to do by using Wasp's Auth UI feature.
When to use Wasp
Wasp is addressing the same core problems that typical web app frameworks are addressing, and it in big part looks, swims and quacks like a web app framework.
Best used for
building full-stack web apps (like e.g. Airbnb or Asana)
quickly starting a web app with industry best practices
to be used alongside modern web dev stack (currently supported React and Node)
Avoid using Wasp for
building static/presentational websites
to be used as a no-code solution
to be a solve-it-all tool in a single language
Wasp is a DSL
note
You don't need to know what a DSL is to use Wasp, but if you are curious, you can read more about it below.
Wasp does not match typical expectations of a web app framework: it is not a set of libraries, it is instead a programming language that understands your code and can do a lot of things for you.
Wasp is a programming language, but a specific kind: it is specialized for a single purpose: building modern web applications. We call such languages DSLs (Domain Specific Language).
Other examples of DSLs that are often used today are e.g. SQL for databases and HTML for web page layouts. The main advantage and reason why DSLs exist is that they need to do only one task (e.g. database queries) so they can do it well and provide the best possible experience for the developer.
The same idea stands behind Wasp - a language that will allow developers to build modern web applications with 10x less code and less stack-specific knowledge. | https://wasp-lang.dev/docs/0.11.8 |
|
1 | 200 | 2024-04-23T15:12:36.917Z | https://wasp-lang.dev/docs/quick-start | https://wasp-lang.dev/docs | browser | ## Quick Start
## Installation[](#installation "Direct link to Installation")
Welcome, new Waspeteer 🐝!
Let's create and run our first Wasp app in 3 short steps:
1. **To install Wasp on Linux / OSX / WSL (Windows), open your terminal and run:**
```
curl -sSL https://get.wasp-lang.dev/installer.sh | sh
```
ℹ️ Wasp requires Node.js and will warn you if it is missing: check below for [more details](#requirements).
2. **Then, create a new app by running:**
3. **Finally, run the app:**
```
cd <my-project-name>wasp start
```
That's it 🎉 You have successfully created and served a new full-stack web app at [http://localhost:3000](http://localhost:3000/) and Wasp is serving both frontend and backend for you.
Something Unclear?
Check [More Details](#more-details) section below if anything went wrong with the installation, or if you have additional questions.
Want an even faster start?
Try out [Wasp AI](https://wasp-lang.dev/docs/wasp-ai/creating-new-app) 🤖 to generate a new Wasp app in minutes just from a title and short description!
Try Wasp Without Installing 🤔?
Give Wasp a spin in the browser without any setup by running our [Wasp Template for Gitpod](https://github.com/wasp-lang/gitpod-template)
### What next?[](#what-next "Direct link to What next?")
* 👉 **Check out the [Todo App tutorial](https://wasp-lang.dev/docs/tutorial/create), which will take you through all the core features of Wasp!** 👈
* [Setup your editor](https://wasp-lang.dev/docs/editor-setup) for working with Wasp.
* Join us on [Discord](https://discord.gg/rzdnErX)! Any feedback or questions you have, we are there for you.
* Follow Wasp development by subscribing to our newsletter: [https://wasp-lang.dev/#signup](https://wasp-lang.dev/#signup) . We usually send 1 per month, and [Matija](https://github.com/matijaSos) does his best to unleash his creativity to make them engaging and fun to read :D!
* * *
## More details[](#more-details "Direct link to More details")
### Requirements[](#requirements "Direct link to Requirements")
You must have Node.js (and NPM) installed on your machine and available in `PATH`. A version of Node.js must be >= 18.
If you need it, we recommend using [nvm](https://github.com/nvm-sh/nvm) for managing your Node.js installation version(s).
A quick guide on installing/using nvm
### Installation[](#installation-1 "Direct link to Installation")
* Linux / macOS
* Windows
* From source
Open your terminal and run:
```
curl -sSL https://get.wasp-lang.dev/installer.sh | sh
```
Running Wasp on Mac with Mx chip (arm64)
**Experiencing the 'Bad CPU type in executable' issue on a device with arm64 (Apple Silicon)?** Given that the wasp binary is built for x86 and not for arm64 (Apple Silicon), you'll need to install [Rosetta on your Mac](https://support.apple.com/en-us/HT211861) if you are using a Mac with Mx (M1, M2, ...). Rosetta is a translation process that enables users to run applications designed for x86 on arm64 (Apple Silicon). To install Rosetta, run the following command in your terminal
```
softwareupdate --install-rosetta
```
Once Rosetta is installed, you should be able to run Wasp without any issues. | null | https://wasp-lang.dev/docs/quick-start | Installation | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecade7a434bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:12:33 GMT | Tue, 23 Apr 2024 15:22:33 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=fU2TKVqUoN7j4JOdDJc05TglhAXnu0G93V03pnl7IajFzp2OpN0ftA3xEwmXGdPXCaQjoU%2FRN8R%2FcDSfqbUR%2BQd8peTQRj0%2FmnfWjnYzb21NqJ4hsWcY3zoeBetcpUxh"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | c67ce5eb289daca68fa242e185f03023e2301b91 | h2 | DCDE:173D:D35F4:102217:6627CFDB | null | MISS | cache-nyc-kteb1890087-NYC | S1713885153.050156, VS0, VE20 | null | null | en | og:image | https://wasp-lang.dev/docs/quick-start | og:url | Quick Start | Wasp | og:title | Installation | og:description | Quick Start | Wasp | null | Quick Start
Installation
Welcome, new Waspeteer 🐝!
Let's create and run our first Wasp app in 3 short steps:
To install Wasp on Linux / OSX / WSL (Windows), open your terminal and run:
curl -sSL https://get.wasp-lang.dev/installer.sh | sh
ℹ️ Wasp requires Node.js and will warn you if it is missing: check below for more details.
Then, create a new app by running:
Finally, run the app:
cd <my-project-name>
wasp start
That's it 🎉 You have successfully created and served a new full-stack web app at http://localhost:3000 and Wasp is serving both frontend and backend for you.
Something Unclear?
Check More Details section below if anything went wrong with the installation, or if you have additional questions.
Want an even faster start?
Try out Wasp AI 🤖 to generate a new Wasp app in minutes just from a title and short description!
Try Wasp Without Installing 🤔?
Give Wasp a spin in the browser without any setup by running our Wasp Template for Gitpod
What next?
👉 Check out the Todo App tutorial, which will take you through all the core features of Wasp! 👈
Setup your editor for working with Wasp.
Join us on Discord! Any feedback or questions you have, we are there for you.
Follow Wasp development by subscribing to our newsletter: https://wasp-lang.dev/#signup . We usually send 1 per month, and Matija does his best to unleash his creativity to make them engaging and fun to read :D!
More details
Requirements
You must have Node.js (and NPM) installed on your machine and available in PATH. A version of Node.js must be >= 18.
If you need it, we recommend using nvm for managing your Node.js installation version(s).
A quick guide on installing/using nvm
Installation
Linux / macOS
Windows
From source
Open your terminal and run:
curl -sSL https://get.wasp-lang.dev/installer.sh | sh
Running Wasp on Mac with Mx chip (arm64)
Experiencing the 'Bad CPU type in executable' issue on a device with arm64 (Apple Silicon)? Given that the wasp binary is built for x86 and not for arm64 (Apple Silicon), you'll need to install Rosetta on your Mac if you are using a Mac with Mx (M1, M2, ...). Rosetta is a translation process that enables users to run applications designed for x86 on arm64 (Apple Silicon). To install Rosetta, run the following command in your terminal
softwareupdate --install-rosetta
Once Rosetta is installed, you should be able to run Wasp without any issues. | https://wasp-lang.dev/docs/quick-start |
|
1 | 200 | 2024-04-23T15:12:37.057Z | https://wasp-lang.dev/docs/editor-setup | https://wasp-lang.dev/docs | browser | ## Editor Setup
note
This page assumes you have already installed Wasp. If you do not have Wasp installed yet, check out the [Quick Start](https://wasp-lang.dev/docs/quick-start) guide.
Wasp comes with the Wasp language server, which gives supported editors powerful support and integration with the language.
## VSCode[](#vscode "Direct link to VSCode")
Currently, Wasp only supports integration with VSCode. Install the [Wasp language extension](https://marketplace.visualstudio.com/items?itemName=wasp-lang.wasp) to get syntax highlighting and integration with the Wasp language server.
The extension enables:
* syntax highlighting for `.wasp` files
* scaffolding of new project files
* code completion
* diagnostics (errors and warnings)
* go to definition
and more! | null | https://wasp-lang.dev/docs/editor-setup | This page assumes you have already installed Wasp. If you do not have Wasp installed yet, check out the Quick Start guide. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecae2df654bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:12:33 GMT | Tue, 23 Apr 2024 15:22:33 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=QZHPrb5EJWuJiGVKAISO9W8V6ZPjAbKMwzlWWXkiHxvMN6cgKL28APlb2gTSy1Fm4sOzaBaPBv1v6S1Ki8QGHAE1jyKCdGB%2BUvbiIDbp7HSkI8lg9CRovG6vb%2F6%2FFsbf"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 47c5be94623d8bc35b5afe59687bebc6127674f7 | h2 | AC82:1736:587160:6A3728:6627CFDC | null | MISS | cache-nyc-kteb1890087-NYC | S1713885154.747935, VS0, VE17 | null | null | en | og:image | https://wasp-lang.dev/docs/editor-setup | og:url | Editor Setup | Wasp | og:title | This page assumes you have already installed Wasp. If you do not have Wasp installed yet, check out the Quick Start guide. | og:description | Editor Setup | Wasp | null | Editor Setup
note
This page assumes you have already installed Wasp. If you do not have Wasp installed yet, check out the Quick Start guide.
Wasp comes with the Wasp language server, which gives supported editors powerful support and integration with the language.
VSCode
Currently, Wasp only supports integration with VSCode. Install the Wasp language extension to get syntax highlighting and integration with the Wasp language server.
The extension enables:
syntax highlighting for .wasp files
scaffolding of new project files
code completion
diagnostics (errors and warnings)
go to definition
and more! | https://wasp-lang.dev/docs/editor-setup |
|
1 | 200 | 2024-04-23T15:12:47.712Z | https://wasp-lang.dev/docs/tutorial/create | https://wasp-lang.dev/docs | browser | info
You'll need to have the latest version of Wasp installed locally to follow this tutorial. If you haven't installed it yet, check out the [QuickStart](https://wasp-lang.dev/docs/quick-start) guide!
In this section, we'll guide you through the process of creating a simple Todo app with Wasp. In the process, we'll take you through the most important and useful features of Wasp.
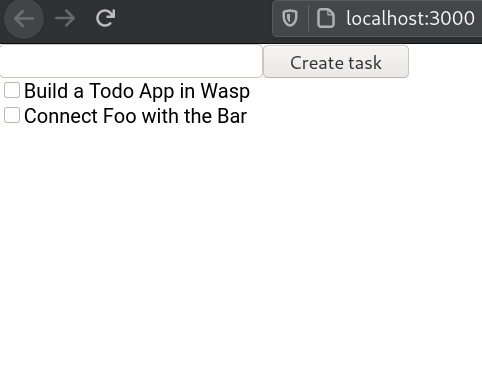
If you get stuck at any point (or just want to chat), reach out to us on [Discord](https://discord.gg/rzdnErX) and we will help you!
You can find the complete code of the app we're about to build [here](https://github.com/wasp-lang/wasp/tree/release/examples/tutorials/TodoApp).
## Creating a Project[](#creating-a-project "Direct link to Creating a Project")
To setup a new Wasp project, run the following command in your terminal
Enter the newly created directory and start the development server:
note
`wasp start` will take a bit of time to start the server the first time you run it in a new project.
You will see log messages from the client, server, and database setting themselves up. When everything is ready, a new tab should open in your browser at `http://localhost:3000` with a simple placeholder page:
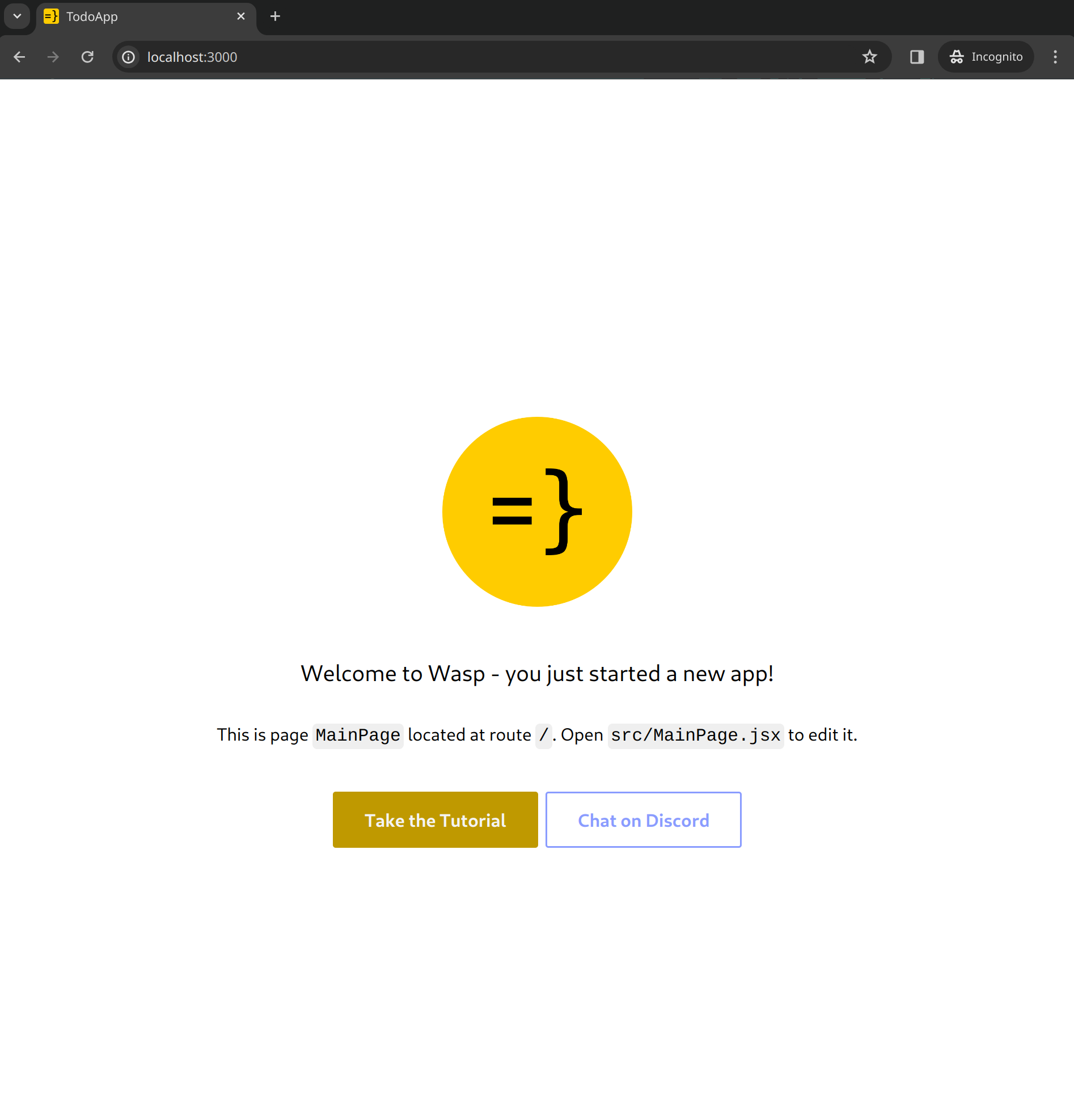
Wasp has generated for you the full front-end and back-end code the app! Next, we'll take a closer look at how the project is structured. | null | https://wasp-lang.dev/docs/tutorial/create | You'll need to have the latest version of Wasp installed locally to follow this tutorial. If you haven't installed it yet, check out the QuickStart guide! | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecb1c5a4c4bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:12:42 GMT | Tue, 23 Apr 2024 15:22:42 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=eyTMcZt%2BGH%2BWupEu6N4Ads7TMIFR3L50qp1wa55mYWE75EKg7dncdJ%2BqytXpmUTrTk0MgjEAN9aTQLO1VORNv%2FtJwAkIevqZ6aP0lETuFYTj5Cm1xX9NS9Y9Jf3eiEiM"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 1da3cdaf9bd3ef0f9eb33730b71574a562b06d83 | h2 | DCDE:173D:D3CEC:102A2D:6627CFE6 | null | MISS | cache-nyc-kteb1890087-NYC | S1713885163.945071, VS0, VE15 | null | null | en | og:image | https://wasp-lang.dev/docs/tutorial/create | og:url | 1. Creating a New Project | Wasp | og:title | You'll need to have the latest version of Wasp installed locally to follow this tutorial. If you haven't installed it yet, check out the QuickStart guide! | og:description | 1. Creating a New Project | Wasp | null | info
You'll need to have the latest version of Wasp installed locally to follow this tutorial. If you haven't installed it yet, check out the QuickStart guide!
In this section, we'll guide you through the process of creating a simple Todo app with Wasp. In the process, we'll take you through the most important and useful features of Wasp.
If you get stuck at any point (or just want to chat), reach out to us on Discord and we will help you!
You can find the complete code of the app we're about to build here.
Creating a Project
To setup a new Wasp project, run the following command in your terminal
Enter the newly created directory and start the development server:
note
wasp start will take a bit of time to start the server the first time you run it in a new project.
You will see log messages from the client, server, and database setting themselves up. When everything is ready, a new tab should open in your browser at http://localhost:3000 with a simple placeholder page:
Wasp has generated for you the full front-end and back-end code the app! Next, we'll take a closer look at how the project is structured. | https://wasp-lang.dev/docs/tutorial/create |
|
1 | 200 | 2024-04-23T15:12:49.312Z | https://wasp-lang.dev/docs/tutorial/project-structure | https://wasp-lang.dev/docs | browser | ## 2\. Project Structure
After creating a new Wasp project, you'll get a file structure that looks like this:
```
.├── .gitignore├── main.wasp # Your Wasp code goes here.├── package.json # Your dependencies and project info go here.├── package-lock.json├── public # Your static files (e.g., images, favicon) go here.├── src # Your source code (TS/JS/CSS/HTML) goes here.│ ├── Main.css│ ├── MainPage.jsx│ ├── vite-env.d.ts│ └── waspLogo.png├── tsconfig.json├── vite.config.ts├── .waspignore└── .wasproot
```
By _your code_, we mean the _"the code you write"_, as opposed to the code generated by Wasp. Wasp allows you to organize and structure your code however you think is best - there's no need to separate client files and server files into different directories.
note
We'd normally recommend organizing code by features (i.e., vertically).
However, since this tutorial contains only a handful of files, there's no need for fancy organization. We'll keep it simple by placing everything in the root `src` directory.
Many other files (e.g., `tsconfig.json`, `vite-env.d.ts`, `.wasproot`, etc.) help Wasp and the IDE improve your development experience with autocompletion, IntelliSense, and error reporting.
The `vite.config.ts` file is used to configure [Vite](https://vitejs.dev/guide/), Wasp's build tool of choice. We won't be configuring Vite in this tutorial, so you can safely ignore the file. Still, if you ever end up wanting more control over Vite, you'll find everything you need to know in [custom Vite config docs](https://wasp-lang.dev/docs/project/custom-vite-config).
There's no need to spend more time discussing all the helper files. They'll silently do their job in the background and let you focus on building your app.
TypeScript Support
Wasp supports TypeScript out of the box, but you are free to choose between or mix JavaScript and TypeScript as you see fit.
We'll provide you with both JavaScript and TypeScript code in this tutorial. Code blocks will have a toggle to switch between vanilla JavaScript and TypeScript.
The most important file in the project is `main.wasp`. Wasp uses the configuration within it to perform its magic. Based on what you write, it generates a bunch of code for your database, server-client communication, React routing, and more.
Let's take a closer look at `main.wasp`
## `main.wasp`[](#mainwasp "Direct link to mainwasp")
`main.wasp` is your app's definition file. It defines the app's central components and helps Wasp to do a lot of the legwork for you.
The file is a list of _declarations_. Each declaration defines a part of your app.
The default `main.wasp` file generated with `wasp new` on the previous page looks like this:
* JavaScript
* TypeScript
main.wasp
```
app TodoApp { wasp: { version: "^0.13.0" // Pins the version of Wasp to use. }, title: "TodoApp" // Used as the browser tab title. Note that all strings in Wasp are double quoted!}route RootRoute { path: "/", to: MainPage }page MainPage { // We specify that the React implementation of the page is exported from // `src/MainPage.jsx`. This statement uses standard JS import syntax. // Use `@src` to reference files inside the `src` folder. component: import { MainPage } from "@src/MainPage"}
```
This file uses three declaration types:
* **app**: Top-level configuration information about your app.
* **route**: Describes which path each page should be accessible from.
* **page**: Defines a web page and the React component that gets rendered when the page is loaded.
In the next section, we'll explore how **route** and **page** work together to build your web app. | null | https://wasp-lang.dev/docs/tutorial/project-structure | After creating a new Wasp project, you'll get a file structure that looks like this: | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecb265c924bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:12:44 GMT | Tue, 23 Apr 2024 15:22:44 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=sPACfgkhL04Y7xVtOQqbA%2B2fXlJox2u%2Fcn7aVr0oa1GemihAJ4tCAXt1VOeuK6i%2BkbEkLxS0BYNwKjRC%2Bd0OIRDyML0SkUZ0Ii%2BYcOMgZ4OVSddBZrNFkr5FcNAtvymH"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 49ac15133e7cbea9c897b42d2006d185269be735 | h2 | D382:180046:3C276BC:472CB83:6627CFEC | HIT | MISS | cache-nyc-kteb1890087-NYC | S1713885165.543913, VS0, VE13 | null | null | en | og:image | https://wasp-lang.dev/docs/tutorial/project-structure | og:url | 2. Project Structure | Wasp | og:title | After creating a new Wasp project, you'll get a file structure that looks like this: | og:description | 2. Project Structure | Wasp | null | 2. Project Structure
After creating a new Wasp project, you'll get a file structure that looks like this:
.
├── .gitignore
├── main.wasp # Your Wasp code goes here.
├── package.json # Your dependencies and project info go here.
├── package-lock.json
├── public # Your static files (e.g., images, favicon) go here.
├── src # Your source code (TS/JS/CSS/HTML) goes here.
│ ├── Main.css
│ ├── MainPage.jsx
│ ├── vite-env.d.ts
│ └── waspLogo.png
├── tsconfig.json
├── vite.config.ts
├── .waspignore
└── .wasproot
By your code, we mean the "the code you write", as opposed to the code generated by Wasp. Wasp allows you to organize and structure your code however you think is best - there's no need to separate client files and server files into different directories.
note
We'd normally recommend organizing code by features (i.e., vertically).
However, since this tutorial contains only a handful of files, there's no need for fancy organization. We'll keep it simple by placing everything in the root src directory.
Many other files (e.g., tsconfig.json, vite-env.d.ts, .wasproot, etc.) help Wasp and the IDE improve your development experience with autocompletion, IntelliSense, and error reporting.
The vite.config.ts file is used to configure Vite, Wasp's build tool of choice. We won't be configuring Vite in this tutorial, so you can safely ignore the file. Still, if you ever end up wanting more control over Vite, you'll find everything you need to know in custom Vite config docs.
There's no need to spend more time discussing all the helper files. They'll silently do their job in the background and let you focus on building your app.
TypeScript Support
Wasp supports TypeScript out of the box, but you are free to choose between or mix JavaScript and TypeScript as you see fit.
We'll provide you with both JavaScript and TypeScript code in this tutorial. Code blocks will have a toggle to switch between vanilla JavaScript and TypeScript.
The most important file in the project is main.wasp. Wasp uses the configuration within it to perform its magic. Based on what you write, it generates a bunch of code for your database, server-client communication, React routing, and more.
Let's take a closer look at main.wasp
main.wasp
main.wasp is your app's definition file. It defines the app's central components and helps Wasp to do a lot of the legwork for you.
The file is a list of declarations. Each declaration defines a part of your app.
The default main.wasp file generated with wasp new on the previous page looks like this:
JavaScript
TypeScript
main.wasp
app TodoApp {
wasp: {
version: "^0.13.0" // Pins the version of Wasp to use.
},
title: "TodoApp" // Used as the browser tab title. Note that all strings in Wasp are double quoted!
}
route RootRoute { path: "/", to: MainPage }
page MainPage {
// We specify that the React implementation of the page is exported from
// `src/MainPage.jsx`. This statement uses standard JS import syntax.
// Use `@src` to reference files inside the `src` folder.
component: import { MainPage } from "@src/MainPage"
}
This file uses three declaration types:
app: Top-level configuration information about your app.
route: Describes which path each page should be accessible from.
page: Defines a web page and the React component that gets rendered when the page is loaded.
In the next section, we'll explore how route and page work together to build your web app. | https://wasp-lang.dev/docs/tutorial/project-structure |
|
1 | 200 | 2024-04-23T15:13:00.817Z | https://wasp-lang.dev/docs/tutorial/pages | https://wasp-lang.dev/docs | browser | ## 3\. Pages & Routes
In the default `main.wasp` file created by `wasp new`, there is a **page** and a **route** declaration:
* JavaScript
* TypeScript
main.wasp
```
route RootRoute { path: "/", to: MainPage }page MainPage { // We specify that the React implementation of the page is exported from // `src/MainPage.jsx`. This statement uses standard JS import syntax. // Use `@src` to reference files inside the `src` folder. component: import { MainPage } from "@src/MainPage"}
```
Together, these declarations tell Wasp that when a user navigates to `/`, it should render the named export from `src/MainPage.tsx`.
## The MainPage Component[](#the-mainpage-component "Direct link to The MainPage Component")
Let's take a look at the React component referenced by the page declaration:
* JavaScript
* TypeScript
src/MainPage.jsx
```
import waspLogo from './waspLogo.png'import './Main.css'export const MainPage = () => { // ...}
```
This is a regular functional React component. It also uses the CSS file and a logo image that sit next to it in the `src` folder.
That is all the code you need! Wasp takes care of everything else necessary to define, build, and run the web app.
tip
`wasp start` automatically picks up the changes you make and restarts the app, so keep it running in the background.
## Adding a Second Page[](#adding-a-second-page "Direct link to Adding a Second Page")
To add more pages, you can create another set of **page** and **route** declarations. You can even add parameters to the URL path, using the same syntax as [React Router](https://reactrouter.com/web/). Let's test this out by adding a new page:
* JavaScript
* TypeScript
main.wasp
```
route HelloRoute { path: "/hello/:name", to: HelloPage }page HelloPage { component: import { HelloPage } from "@src/HelloPage"}
```
When a user visits `/hello/their-name`, Wasp will render the component exported from `src/HelloPage.tsx` and pass the URL parameter the same way as in React Router:
* JavaScript
* TypeScript
src/HelloPage.jsx
```
export const HelloPage = (props) => { return <div>Here's {props.match.params.name}!</div>}
```
Now you can visit `/hello/johnny` and see "Here's johnny!"
## Cleaning Up[](#cleaning-up "Direct link to Cleaning Up")
Now that you've seen how Wasp deals with Routes and Pages, it's finally time to build the Todo app.
Start by cleaning up the starter project and removing unnecessary code and files.
First, remove most of the code from the `MainPage` component:
* JavaScript
* TypeScript
src/MainPage.jsx
```
export const MainPage = () => { return <div>Hello world!</div>}
```
At this point, the main page should look like this:
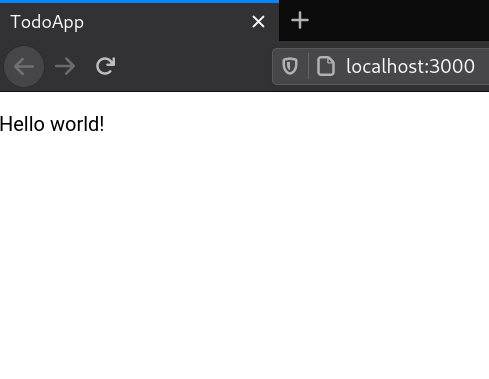
You can now delete redundant files: `src/Main.css`, `src/waspLogo.png`, and `src/HelloPage.tsx` (we won't need this page for the rest of the tutorial).
Since `src/HelloPage.tsx` no longer exists, remove its `route` and `page` declarations from the `main.wasp` file.
Your Wasp file should now look like this:
* JavaScript
* TypeScript
main.wasp
```
app TodoApp { wasp: { version: "^0.13.0" }, title: "TodoApp"}route RootRoute { path: "/", to: MainPage }page MainPage { component: import { MainPage } from "@src/MainPage"}
```
Excellent work!
You now have a basic understanding of Wasp and are ready to start building your TodoApp. We'll implement the app's core features in the following sections. | null | https://wasp-lang.dev/docs/tutorial/pages | In the default main.wasp file created by wasp new, there is a page and a route declaration: | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecb6d9cb04bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:12:55 GMT | Tue, 23 Apr 2024 15:22:55 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=hleH5jjx%2BmjSDRX1YXkd0%2FHDCs1snqLRdRLaPD3F7ez2luqbtDRU2UKxYxbvh0S0fGIeNMeGa%2B7MbpI0RgOtkdRPJfqwbXA8%2BMEgr%2BxcG2l9XDVpOva8OdJAYb35qQRT"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 28ba51b678b151877f02911abb924070ac4c9ffb | h2 | D65C:39E569:39EF43E:44CED7B:6627CFF7 | null | MISS | cache-nyc-kteb1890087-NYC | S1713885176.951885, VS0, VE15 | null | null | en | og:image | https://wasp-lang.dev/docs/tutorial/pages | og:url | 3. Pages & Routes | Wasp | og:title | In the default main.wasp file created by wasp new, there is a page and a route declaration: | og:description | 3. Pages & Routes | Wasp | null | 3. Pages & Routes
In the default main.wasp file created by wasp new, there is a page and a route declaration:
JavaScript
TypeScript
main.wasp
route RootRoute { path: "/", to: MainPage }
page MainPage {
// We specify that the React implementation of the page is exported from
// `src/MainPage.jsx`. This statement uses standard JS import syntax.
// Use `@src` to reference files inside the `src` folder.
component: import { MainPage } from "@src/MainPage"
}
Together, these declarations tell Wasp that when a user navigates to /, it should render the named export from src/MainPage.tsx.
The MainPage Component
Let's take a look at the React component referenced by the page declaration:
JavaScript
TypeScript
src/MainPage.jsx
import waspLogo from './waspLogo.png'
import './Main.css'
export const MainPage = () => {
// ...
}
This is a regular functional React component. It also uses the CSS file and a logo image that sit next to it in the src folder.
That is all the code you need! Wasp takes care of everything else necessary to define, build, and run the web app.
tip
wasp start automatically picks up the changes you make and restarts the app, so keep it running in the background.
Adding a Second Page
To add more pages, you can create another set of page and route declarations. You can even add parameters to the URL path, using the same syntax as React Router. Let's test this out by adding a new page:
JavaScript
TypeScript
main.wasp
route HelloRoute { path: "/hello/:name", to: HelloPage }
page HelloPage {
component: import { HelloPage } from "@src/HelloPage"
}
When a user visits /hello/their-name, Wasp will render the component exported from src/HelloPage.tsx and pass the URL parameter the same way as in React Router:
JavaScript
TypeScript
src/HelloPage.jsx
export const HelloPage = (props) => {
return <div>Here's {props.match.params.name}!</div>
}
Now you can visit /hello/johnny and see "Here's johnny!"
Cleaning Up
Now that you've seen how Wasp deals with Routes and Pages, it's finally time to build the Todo app.
Start by cleaning up the starter project and removing unnecessary code and files.
First, remove most of the code from the MainPage component:
JavaScript
TypeScript
src/MainPage.jsx
export const MainPage = () => {
return <div>Hello world!</div>
}
At this point, the main page should look like this:
You can now delete redundant files: src/Main.css, src/waspLogo.png, and src/HelloPage.tsx (we won't need this page for the rest of the tutorial).
Since src/HelloPage.tsx no longer exists, remove its route and page declarations from the main.wasp file.
Your Wasp file should now look like this:
JavaScript
TypeScript
main.wasp
app TodoApp {
wasp: {
version: "^0.13.0"
},
title: "TodoApp"
}
route RootRoute { path: "/", to: MainPage }
page MainPage {
component: import { MainPage } from "@src/MainPage"
}
Excellent work!
You now have a basic understanding of Wasp and are ready to start building your TodoApp. We'll implement the app's core features in the following sections. | https://wasp-lang.dev/docs/tutorial/pages |
|
1 | 200 | 2024-04-23T15:13:03.445Z | https://wasp-lang.dev/docs/tutorial/entities | https://wasp-lang.dev/docs | browser | ```
// ...entity Task {=psl id Int @id @default(autoincrement()) description String isDone Boolean @default(false)psl=}
``` | null | https://wasp-lang.dev/docs/tutorial/entities | Entities are one of the most important concepts in Wasp and are how you define what gets stored in the database. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecb84bddf4bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:12:59 GMT | Tue, 23 Apr 2024 15:22:59 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=4IqQ3HCNK7lGV%2FlPYh9a58UJt6LT1U%2BQYjpdiEU3MVMjhSH8OawIUbknsjwmjX30jk6pkB%2FGeYZPFmFIuCozS7OOFgc7zQtSi58NXNTXWLiQ3o6jWiPn6x0toSa3Kx5k"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 4b5f54d0c4d67ec6e5a4c17132510dd6be2fd795 | h2 | 2E70:2405A0:3CC8093:47A7A2A:6627CFFA | null | MISS | cache-nyc-kteb1890099-NYC | S1713885180.662911, VS0, VE21 | null | null | en | og:image | https://wasp-lang.dev/docs/tutorial/entities | og:url | 4. Database Entities | Wasp | og:title | Entities are one of the most important concepts in Wasp and are how you define what gets stored in the database. | og:description | 4. Database Entities | Wasp | null | // ...
entity Task {=psl
id Int @id @default(autoincrement())
description String
isDone Boolean @default(false)
psl=} | https://wasp-lang.dev/docs/tutorial/entities |
|
1 | 200 | 2024-04-23T15:13:03.628Z | https://wasp-lang.dev/docs/tutorial/queries | https://wasp-lang.dev/docs | browser | ## 5\. Querying the Database
We want to know which tasks we need to do, so let's list them!
The primary way of working with Entities in Wasp is with [Queries and Actions](https://wasp-lang.dev/docs/data-model/operations/overview), collectively known as **_Operations_**.
Queries are used to read an entity, while Actions are used to create, modify, and delete entities. Since we want to list the tasks, we'll want to use a Query.
To list the tasks, you must:
1. Create a Query that fetches the tasks from the database.
2. Update the `MainPage.tsx` to use that Query and display the results.
## Defining the Query[](#defining-the-query "Direct link to Defining the Query")
We'll create a new Query called `getTasks`. We'll need to declare the Query in the Wasp file and write its implementation in .
### Declaring a Query[](#declaring-a-query "Direct link to Declaring a Query")
We need to add a **query** declaration to `main.wasp` so that Wasp knows it exists:
* JavaScript
* TypeScript
main.wasp
```
// ...query getTasks { // Specifies where the implementation for the query function is. // The path `@src/queries` resolves to `src/queries.js`. // No need to specify an extension. fn: import { getTasks } from "@src/queries", // Tell Wasp that this query reads from the `Task` entity. Wasp will // automatically update the results of this query when tasks are modified. entities: [Task]}
```
### Implementing a Query[](#implementing-a-query "Direct link to Implementing a Query")
* JavaScript
* TypeScript
src/queries.js
```
export const getTasks = async (args, context) => { return context.entities.Task.findMany({ orderBy: { id: 'asc' }, })}
```
Query function parameters:
* `args: object`
The arguments the caller passes to the Query.
* `context`
An object with extra information injected by Wasp. Its type depends on the Query declaration.
Since the Query declaration in `main.wasp` says that the `getTasks` Query uses `Task` entity, Wasp injected a [Prisma client](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-client/crud) for the `Task` entity as `context.entities.Task` - we used it above to fetch all the tasks from the database.
info
Queries and Actions are NodeJS functions executed on the server.
## Invoking the Query On the Frontend[](#invoking-the-query-on-the-frontend "Direct link to Invoking the Query On the Frontend")
While we implement Queries on the server, Wasp generates client-side functions that automatically take care of serialization, network calls, and cache invalidation, allowing you to call the server code like it's a regular function.
This makes it easy for us to use the `getTasks` Query we just created in our React component:
* JavaScript
* TypeScript
src/MainPage.jsx
```
import { getTasks, useQuery } from 'wasp/client/operations'export const MainPage = () => { const { data: tasks, isLoading, error } = useQuery(getTasks) return ( <div> {tasks && <TasksList tasks={tasks} />} {isLoading && 'Loading...'} {error && 'Error: ' + error} </div> )}const TaskView = ({ task }) => { return ( <div> <input type="checkbox" id={String(task.id)} checked={task.isDone} /> {task.description} </div> )}const TasksList = ({ tasks }) => { if (!tasks?.length) return <div>No tasks</div> return ( <div> {tasks.map((task, idx) => ( <TaskView task={task} key={idx} /> ))} </div> )}
```
Most of this code is regular React, the only exception being the special `wasp` imports:
We could have called the Query directly using `getTasks()`, but the `useQuery` hook makes it reactive: React will re-render the component every time the Query changes. Remember that Wasp automatically refreshes Queries whenever the data is modified.
With these changes, you should be seeing the text "No tasks" on the screen:
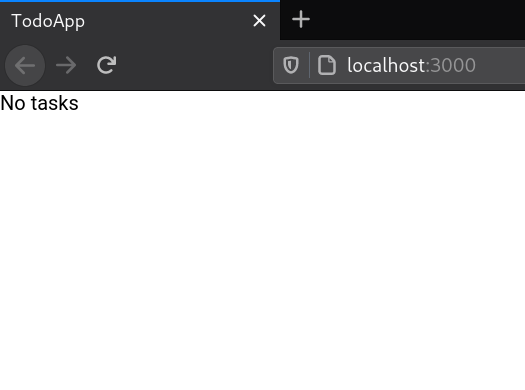
We'll create a form to add tasks in the next step 🪄 | null | https://wasp-lang.dev/docs/tutorial/queries | We want to know which tasks we need to do, so let's list them! | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecb73e8ec4bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:12:57 GMT | Tue, 23 Apr 2024 15:22:56 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=qLvNkVdaAVxanCN2FIMN8vnWpfBkK2t1Uk0gZqcVWuEOc6XZ3TGYxOloH6PIVnkZ5CUk5b7o5RnYKYD%2BH2iTX1bM9H65yPgq4Z17TCEWLRg5KtPiTz4AY8Vk%2FYxLo%2F4c"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 597d5a4eb44bb131305963a05405d34c5416bb81 | h2 | 5FBE:171D:735E53:88D80E:6627CFF8 | null | MISS | cache-nyc-kteb1890087-NYC | S1713885177.954366, VS0, VE48 | null | null | en | og:image | https://wasp-lang.dev/docs/tutorial/queries | og:url | 5. Querying the Database | Wasp | og:title | We want to know which tasks we need to do, so let's list them! | og:description | 5. Querying the Database | Wasp | null | 5. Querying the Database
We want to know which tasks we need to do, so let's list them!
The primary way of working with Entities in Wasp is with Queries and Actions, collectively known as Operations.
Queries are used to read an entity, while Actions are used to create, modify, and delete entities. Since we want to list the tasks, we'll want to use a Query.
To list the tasks, you must:
Create a Query that fetches the tasks from the database.
Update the MainPage.tsx to use that Query and display the results.
Defining the Query
We'll create a new Query called getTasks. We'll need to declare the Query in the Wasp file and write its implementation in .
Declaring a Query
We need to add a query declaration to main.wasp so that Wasp knows it exists:
JavaScript
TypeScript
main.wasp
// ...
query getTasks {
// Specifies where the implementation for the query function is.
// The path `@src/queries` resolves to `src/queries.js`.
// No need to specify an extension.
fn: import { getTasks } from "@src/queries",
// Tell Wasp that this query reads from the `Task` entity. Wasp will
// automatically update the results of this query when tasks are modified.
entities: [Task]
}
Implementing a Query
JavaScript
TypeScript
src/queries.js
export const getTasks = async (args, context) => {
return context.entities.Task.findMany({
orderBy: { id: 'asc' },
})
}
Query function parameters:
args: object
The arguments the caller passes to the Query.
context
An object with extra information injected by Wasp. Its type depends on the Query declaration.
Since the Query declaration in main.wasp says that the getTasks Query uses Task entity, Wasp injected a Prisma client for the Task entity as context.entities.Task - we used it above to fetch all the tasks from the database.
info
Queries and Actions are NodeJS functions executed on the server.
Invoking the Query On the Frontend
While we implement Queries on the server, Wasp generates client-side functions that automatically take care of serialization, network calls, and cache invalidation, allowing you to call the server code like it's a regular function.
This makes it easy for us to use the getTasks Query we just created in our React component:
JavaScript
TypeScript
src/MainPage.jsx
import { getTasks, useQuery } from 'wasp/client/operations'
export const MainPage = () => {
const { data: tasks, isLoading, error } = useQuery(getTasks)
return (
<div>
{tasks && <TasksList tasks={tasks} />}
{isLoading && 'Loading...'}
{error && 'Error: ' + error}
</div>
)
}
const TaskView = ({ task }) => {
return (
<div>
<input type="checkbox" id={String(task.id)} checked={task.isDone} />
{task.description}
</div>
)
}
const TasksList = ({ tasks }) => {
if (!tasks?.length) return <div>No tasks</div>
return (
<div>
{tasks.map((task, idx) => (
<TaskView task={task} key={idx} />
))}
</div>
)
}
Most of this code is regular React, the only exception being the special wasp imports:
We could have called the Query directly using getTasks(), but the useQuery hook makes it reactive: React will re-render the component every time the Query changes. Remember that Wasp automatically refreshes Queries whenever the data is modified.
With these changes, you should be seeing the text "No tasks" on the screen:
We'll create a form to add tasks in the next step 🪄 | https://wasp-lang.dev/docs/tutorial/queries |
|
1 | 200 | 2024-04-23T15:13:17.505Z | https://wasp-lang.dev/docs/tutorial/actions | https://wasp-lang.dev/docs | browser | ## 6\. Modifying Data
In the previous section, we learned about using Queries to fetch data and only briefly mentioned that Actions can be used to update the database. Let's learn more about Actions so we can add and update tasks in the database.
We have to create:
1. A Wasp Action that creates a new task.
2. A React form that calls that Action when the user creates a task.
## Creating a New Action[](#creating-a-new-action "Direct link to Creating a New Action")
Creating an Action is very similar to creating a Query.
### Declaring an Action[](#declaring-an-action "Direct link to Declaring an Action")
We must first declare the Action in `main.wasp`:
* JavaScript
* TypeScript
main.wasp
```
// ...action createTask { fn: import { createTask } from "@src/actions", entities: [Task]}
```
### Implementing an Action[](#implementing-an-action "Direct link to Implementing an Action")
Let's now define a function for our `createTask` Action:
* JavaScript
* TypeScript
src/actions.js
```
export const createTask = async (args, context) => { return context.entities.Task.create({ data: { description: args.description }, })}
```
tip
We put the function in a new file `src/actions.ts`, but we could have put it anywhere we wanted! There are no limitations here, as long as the declaration in the Wasp file imports it correctly and the file is located within `src` directory.
## Invoking the Action on the Client[](#invoking-the-action-on-the-client "Direct link to Invoking the Action on the Client")
Start by defining a form for creating new tasks.
* JavaScript
* TypeScript
src/MainPage.jsx
```
import { createTask, getTasks, useQuery } from 'wasp/client/operations'// ... MainPage, TaskView, TaskList ...const NewTaskForm = () => { const handleSubmit = async (event) => { event.preventDefault() try { const target = event.target const description = target.description.value target.reset() await createTask({ description }) } catch (err) { window.alert('Error: ' + err.message) } } return ( <form onSubmit={handleSubmit}> <input name="description" type="text" defaultValue="" /> <input type="submit" value="Create task" /> </form> )}
```
Unlike Queries, you can call Actions directly (i.e., without wrapping it with a hook) because we don't need reactivity. The rest is just regular React code.
All that's left now is adding this form to the page component:
* JavaScript
* TypeScript
src/MainPage.jsx
```
import { createTask, getTasks, useQuery} from 'wasp/client/operations'const MainPage = () => { const { data: tasks, isLoading, error } = useQuery(getTasks) return ( <div> <NewTaskForm /> {tasks && <TasksList tasks={tasks} />} {isLoading && 'Loading...'} {error && 'Error: ' + error} </div> )}// ... TaskView, TaskList, NewTaskForm ...
```
Great work!
You now have a form for creating new tasks.
Try creating a "Build a Todo App in Wasp" task and see it appear in the list below. The task is created on the server and saved in the database.
Try refreshing the page or opening it in another browser. You'll see the tasks are still there!
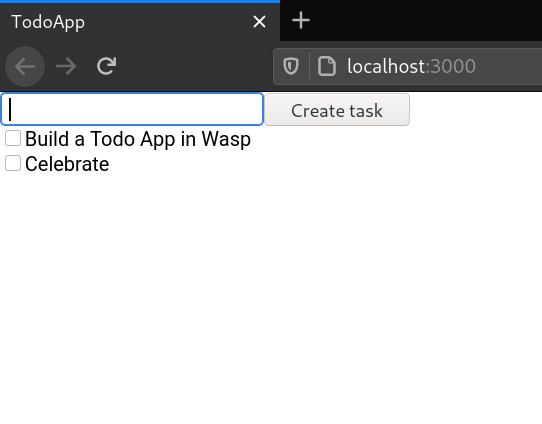
Automatic Query Invalidation
When you create a new task, the list of tasks is automatically updated to display the new task, even though we have not written any code that would do that! Wasp handles these automatic updates under the hood.
When you declared the `getTasks` and `createTask` operations, you specified that they both use the `Task` entity. So when `createTask` is called, Wasp knows that the data `getTasks` fetches may have changed and automatically updates it in the background. This means that **out of the box, Wasp keeps all your queries in sync with any changes made through Actions**.
This behavior is convenient as a default but can cause poor performance in large apps. While there is no mechanism for overriding this behavior yet, it is something that we plan to include in Wasp in the future. This feature is tracked [here](https://github.com/wasp-lang/wasp/issues/63).
## A Second Action[](#a-second-action "Direct link to A Second Action")
Our Todo app isn't finished if you can't mark a task as done.
We'll create a new Action to update a task's status and call it from React whenever a task's checkbox is toggled.
Since we've already created one task together, try to create this one yourself. It should be an Action named `updateTask` that receives the task's `id` and its `isDone` status. You can see our implementation below.
Solution
You can now call `updateTask` from the React component:
* JavaScript
* TypeScript
src/MainPage.jsx
```
// ...import { updateTask, createTask, getTasks, useQuery,} from 'wasp/client/operations'// ... MainPage ...const TaskView = ({ task }) => { const handleIsDoneChange = async (event) => { try { await updateTask({ id: task.id, isDone: event.target.checked, }) } catch (error) { window.alert('Error while updating task: ' + error.message) } } return ( <div> <input type="checkbox" id={String(task.id)} checked={task.isDone} onChange={handleIsDoneChange} /> {task.description} </div> )}// ... TaskList, NewTaskForm ...
```
Awesome! You can now mark this task as done.
It's time to make one final addition to your app: supporting multiple users. | null | https://wasp-lang.dev/docs/tutorial/actions | In the previous section, we learned about using Queries to fetch data and only briefly mentioned that Actions can be used to update the database. Let's learn more about Actions so we can add and update tasks in the database. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecbdf89344bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:13:14 GMT | Tue, 23 Apr 2024 15:23:14 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=IW%2FhAf7JC93cXEoHPUub5bYWIWfqznqe3lCES32F4O%2BX6SX2QHsK7i4TpgNUz%2FdQ7jzw6oZnKQWxtbqvSbpJoBgLi4BIZZXIizPTDAC8UreMZV%2FufJrA5%2F2xnFO3GzeJ"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | a08aa578f5bfe198673dd0ca1cc7c727b0b8590e | h2 | A07A:2405A0:3CC8CCB:47A8813:6627D007 | HIT | MISS | cache-nyc-kteb1890087-NYC | S1713885194.180478, VS0, VE19 | null | null | en | og:image | https://wasp-lang.dev/docs/tutorial/actions | og:url | 6. Modifying Data | Wasp | og:title | In the previous section, we learned about using Queries to fetch data and only briefly mentioned that Actions can be used to update the database. Let's learn more about Actions so we can add and update tasks in the database. | og:description | 6. Modifying Data | Wasp | null | 6. Modifying Data
In the previous section, we learned about using Queries to fetch data and only briefly mentioned that Actions can be used to update the database. Let's learn more about Actions so we can add and update tasks in the database.
We have to create:
A Wasp Action that creates a new task.
A React form that calls that Action when the user creates a task.
Creating a New Action
Creating an Action is very similar to creating a Query.
Declaring an Action
We must first declare the Action in main.wasp:
JavaScript
TypeScript
main.wasp
// ...
action createTask {
fn: import { createTask } from "@src/actions",
entities: [Task]
}
Implementing an Action
Let's now define a function for our createTask Action:
JavaScript
TypeScript
src/actions.js
export const createTask = async (args, context) => {
return context.entities.Task.create({
data: { description: args.description },
})
}
tip
We put the function in a new file src/actions.ts, but we could have put it anywhere we wanted! There are no limitations here, as long as the declaration in the Wasp file imports it correctly and the file is located within src directory.
Invoking the Action on the Client
Start by defining a form for creating new tasks.
JavaScript
TypeScript
src/MainPage.jsx
import {
createTask,
getTasks,
useQuery
} from 'wasp/client/operations'
// ... MainPage, TaskView, TaskList ...
const NewTaskForm = () => {
const handleSubmit = async (event) => {
event.preventDefault()
try {
const target = event.target
const description = target.description.value
target.reset()
await createTask({ description })
} catch (err) {
window.alert('Error: ' + err.message)
}
}
return (
<form onSubmit={handleSubmit}>
<input name="description" type="text" defaultValue="" />
<input type="submit" value="Create task" />
</form>
)
}
Unlike Queries, you can call Actions directly (i.e., without wrapping it with a hook) because we don't need reactivity. The rest is just regular React code.
All that's left now is adding this form to the page component:
JavaScript
TypeScript
src/MainPage.jsx
import {
createTask,
getTasks,
useQuery
} from 'wasp/client/operations'
const MainPage = () => {
const { data: tasks, isLoading, error } = useQuery(getTasks)
return (
<div>
<NewTaskForm />
{tasks && <TasksList tasks={tasks} />}
{isLoading && 'Loading...'}
{error && 'Error: ' + error}
</div>
)
}
// ... TaskView, TaskList, NewTaskForm ...
Great work!
You now have a form for creating new tasks.
Try creating a "Build a Todo App in Wasp" task and see it appear in the list below. The task is created on the server and saved in the database.
Try refreshing the page or opening it in another browser. You'll see the tasks are still there!
Automatic Query Invalidation
When you create a new task, the list of tasks is automatically updated to display the new task, even though we have not written any code that would do that! Wasp handles these automatic updates under the hood.
When you declared the getTasks and createTask operations, you specified that they both use the Task entity. So when createTask is called, Wasp knows that the data getTasks fetches may have changed and automatically updates it in the background. This means that out of the box, Wasp keeps all your queries in sync with any changes made through Actions.
This behavior is convenient as a default but can cause poor performance in large apps. While there is no mechanism for overriding this behavior yet, it is something that we plan to include in Wasp in the future. This feature is tracked here.
A Second Action
Our Todo app isn't finished if you can't mark a task as done.
We'll create a new Action to update a task's status and call it from React whenever a task's checkbox is toggled.
Since we've already created one task together, try to create this one yourself. It should be an Action named updateTask that receives the task's id and its isDone status. You can see our implementation below.
Solution
You can now call updateTask from the React component:
JavaScript
TypeScript
src/MainPage.jsx
// ...
import {
updateTask,
createTask,
getTasks,
useQuery,
} from 'wasp/client/operations'
// ... MainPage ...
const TaskView = ({ task }) => {
const handleIsDoneChange = async (event) => {
try {
await updateTask({
id: task.id,
isDone: event.target.checked,
})
} catch (error) {
window.alert('Error while updating task: ' + error.message)
}
}
return (
<div>
<input
type="checkbox"
id={String(task.id)}
checked={task.isDone}
onChange={handleIsDoneChange}
/>
{task.description}
</div>
)
}
// ... TaskList, NewTaskForm ...
Awesome! You can now mark this task as done.
It's time to make one final addition to your app: supporting multiple users. | https://wasp-lang.dev/docs/tutorial/actions |
|
1 | null | 2024-04-23T15:13:25.755Z | https://wasp-lang.dev/docs/tutorial/auth | https://wasp-lang.dev/docs | http | ## 7\. Adding Authentication
Most modern apps need a way to create and authenticate users. Wasp makes this as easy as possible with its first-class auth support.
To add users to your app, you must:
* Create a `User` Entity.
* Tell Wasp to use the _Username and Password_ authentication.
* Add login and signup pages.
* Update the main page to require authentication.
* Add a relation between `User` and `Task` entities.
* Modify your Queries and Actions so users can only see and modify their tasks.
* Add a logout button.
## Creating a User Entity[](#creating-a-user-entity "Direct link to Creating a User Entity")
Since Wasp manages authentication, it will create [the auth related entities](https://wasp-lang.dev/docs/auth/entities) for you in the background. Nothing to do here!
You must only add the `User` Entity to keep track of who owns which tasks.
main.wasp
```
// ...entity User {=psl id Int @id @default(autoincrement())psl=}
```
## Adding Auth to the Project[](#adding-auth-to-the-project "Direct link to Adding Auth to the Project")
Next, tell Wasp to use full-stack [authentication](https://wasp-lang.dev/docs/auth/overview):
main.wasp
```
app TodoApp { wasp: { version: "^0.13.0" }, title: "TodoApp", auth: { // Tells Wasp which entity to use for storing users. userEntity: User, methods: { // Enable username and password auth. usernameAndPassword: {} }, // We'll see how this is used in a bit. onAuthFailedRedirectTo: "/login" }}// ...
```
Don't forget to update the database schema by running:
By doing this, Wasp will create:
* [Auth UI](https://wasp-lang.dev/docs/auth/ui) with login and signup forms.
* A `logout()` action.
* A React hook `useAuth()`.
* `context.user` for use in Queries and Actions.
info
Wasp also supports authentication using [Google](https://wasp-lang.dev/docs/auth/social-auth/google), [GitHub](https://wasp-lang.dev/docs/auth/social-auth/github), and [email](https://wasp-lang.dev/docs/auth/email), with more on the way!
## Adding Login and Signup Pages[](#adding-login-and-signup-pages "Direct link to Adding Login and Signup Pages")
Wasp creates the login and signup forms for us, but we still need to define the pages to display those forms on. We'll start by declaring the pages in the Wasp file:
* JavaScript
* TypeScript
main.wasp
```
// ...route SignupRoute { path: "/signup", to: SignupPage }page SignupPage { component: import { SignupPage } from "@src/SignupPage"}route LoginRoute { path: "/login", to: LoginPage }page LoginPage { component: import { LoginPage } from "@src/LoginPage"}
```
Great, Wasp now knows these pages exist!
Here's the React code for the pages you've just imported:
* JavaScript
* TypeScript
src/LoginPage.jsx
```
import { Link } from 'react-router-dom'import { LoginForm } from 'wasp/client/auth'export const LoginPage = () => { return ( <div style={{ maxWidth: '400px', margin: '0 auto' }}> <LoginForm /> <br /> <span> I don't have an account yet (<Link to="/signup">go to signup</Link>). </span> </div> )}
```
The signup page is very similar to the login page:
* JavaScript
* TypeScript
src/SignupPage.jsx
```
import { Link } from 'react-router-dom'import { SignupForm } from 'wasp/client/auth'export const SignupPage = () => { return ( <div style={{ maxWidth: '400px', margin: '0 auto' }}> <SignupForm /> <br /> <span> I already have an account (<Link to="/login">go to login</Link>). </span> </div> )}
```
## Update the Main Page to Require Auth[](#update-the-main-page-to-require-auth "Direct link to Update the Main Page to Require Auth")
We don't want users who are not logged in to access the main page, because they won't be able to create any tasks. So let's make the page private by requiring the user to be logged in:
main.wasp
```
// ...page MainPage { authRequired: true, component: import { MainPage } from "@src/MainPage"}
```
Now that auth is required for this page, unauthenticated users will be redirected to `/login`, as we specified with `app.auth.onAuthFailedRedirectTo`.
Additionally, when `authRequired` is `true`, the page's React component will be provided a `user` object as prop.
* JavaScript
* TypeScript
src/MainPage.jsx
```
export const MainPage = ({ user }) => { // Do something with the user // ...}
```
Ok, time to test this out. Navigate to the main page (`/`) of the app. You'll get redirected to `/login`, where you'll be asked to authenticate.
Since we just added users, you don't have an account yet. Go to the signup page and create one. You'll be sent back to the main page where you will now be able to see the TODO list!
Let's check out what the database looks like. Start the Prisma Studio:
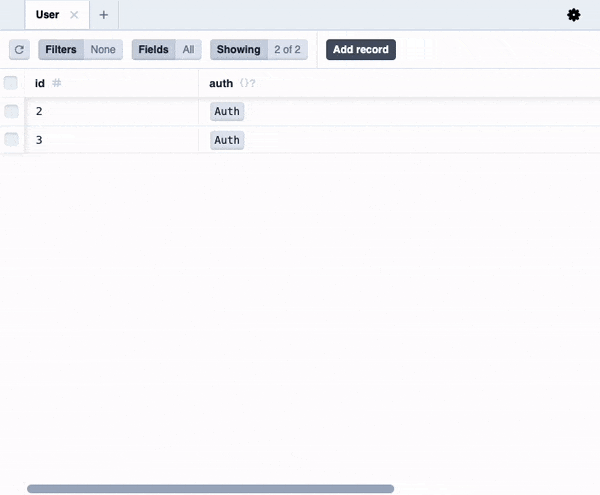
You'll notice that we now have a `User` entity in the database alongside the `Task` entity.
However, you will notice that if you try logging in as different users and creating some tasks, all users share the same tasks. That's because we haven't yet updated the queries and actions to have per-user tasks. Let's do that next.
You might notice some extra Prisma models like `Auth`, `AuthIdentity` and `Session` that Wasp created for us. You don't need to care about these right now, but if you are curious, you can read more about them [here](https://wasp-lang.dev/docs/auth/entities).
## Defining a User-Task Relation[](#defining-a-user-task-relation "Direct link to Defining a User-Task Relation")
First, let's define a one-to-many relation between users and tasks (check the [Prisma docs on relations](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-schema/relations)):
main.wasp
```
// ...entity User {=psl id Int @id @default(autoincrement()) tasks Task[]psl=}entity Task {=psl id Int @id @default(autoincrement()) description String isDone Boolean @default(false) user User? @relation(fields: [userId], references: [id]) userId Int?psl=}// ...
```
As always, you must migrate the database after changing the Entities:
note
We made `user` and `userId` in `Task` optional (via `?`) because that allows us to keep the existing tasks, which don't have a user assigned, in the database.
This isn't recommended because it allows an unwanted state in the database (what is the purpose of the task not belonging to anybody?) and normally we would not make these fields optional.
Instead, we would do a data migration to take care of those tasks, even if it means just deleting them all. However, for this tutorial, for the sake of simplicity, we will stick with this.
## Updating Operations to Check Authentication[](#updating-operations-to-check-authentication "Direct link to Updating Operations to Check Authentication")
Next, let's update the queries and actions to forbid access to non-authenticated users and to operate only on the currently logged-in user's tasks:
* JavaScript
* TypeScript
src/queries.js
```
import { HttpError } from 'wasp/server'export const getTasks = async (args, context) => { if (!context.user) { throw new HttpError(401) } return context.entities.Task.findMany({ where: { user: { id: context.user.id } }, orderBy: { id: 'asc' }, })}
```
* JavaScript
* TypeScript
src/actions.js
```
import { HttpError } from 'wasp/server'export const createTask = async (args, context) => { if (!context.user) { throw new HttpError(401) } return context.entities.Task.create({ data: { description: args.description, user: { connect: { id: context.user.id } }, }, })}export const updateTask = async (args, context) => { if (!context.user) { throw new HttpError(401) } return context.entities.Task.updateMany({ where: { id: args.id, user: { id: context.user.id } }, data: { isDone: args.isDone }, })}
```
note
Due to how Prisma works, we had to convert `update` to `updateMany` in `updateTask` action to be able to specify the user id in `where`.
With these changes, each user should have a list of tasks that only they can see and edit.
Try playing around, adding a few users and some tasks for each of them. Then open the DB studio:
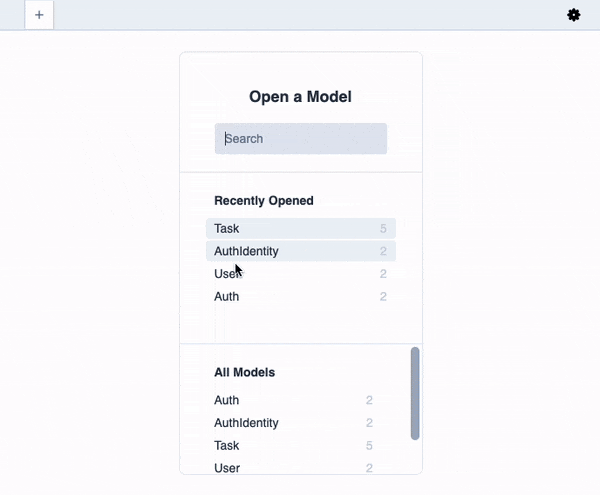
You will see that each user has their tasks, just as we specified in our code!
## Logout Button[](#logout-button "Direct link to Logout Button")
Last, but not least, let's add the logout functionality:
* JavaScript
* TypeScript
src/MainPage.jsx
```
// ...import { logout } from 'wasp/client/auth'//...const MainPage = () => { // ... return ( <div> // ... <button onClick={logout}>Logout</button> </div> )}
```
This is it, we have a working authentication system, and our Todo app is multi-user!
## What's Next?[](#whats-next "Direct link to What's Next?")
We did it 🎉 You've followed along with this tutorial to create a basic Todo app with Wasp.
You should be ready to learn about more complicated features and go more in-depth with the features already covered. Scroll through the sidebar on the left side of the page to see every feature Wasp has to offer. Or, let your imagination run wild and start building your app! ✨
Looking for inspiration?
* Get a jump start on your next project with [Starter Templates](https://wasp-lang.dev/docs/project/starter-templates)
* Make a real-time app with [Web Sockets](https://wasp-lang.dev/docs/advanced/web-sockets)
note
If you notice that some of the features you'd like to have are missing, or have any other kind of feedback, please write to us on [Discord](https://discord.gg/rzdnErX) or create an issue on [Github](https://github.com/wasp-lang/wasp), so we can learn which features to add/improve next 🙏
If you would like to contribute or help to build a feature, let us know! You can find more details on contributing [here](https://wasp-lang.dev/docs/contributing).
Oh, and do [**subscribe to our newsletter**](https://wasp-lang.dev/#signup)! We usually send one per month, and Matija does his best to unleash his creativity to make them engaging and fun to read :D! | null | https://wasp-lang.dev/docs/tutorial/auth | Most modern apps need a way to create and authenticate users. Wasp makes this as easy as possible with its first-class auth support. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecc273d8807dd-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:13:25 GMT | Tue, 23 Apr 2024 15:23:25 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=bZhWgmUk8%2F%2BETb40OJSb3Bl2u9mtB98CFudvEfNVDQLmI%2FQFybvqT4j6yqaKFnq347lfNZdo%2BN%2B2ByYkgCMBMmQfI54iZ7yEbYBmXzPeuJ%2BskfxWnzIWhbcQVEXfxxmY"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 29bf5e68a9b9f54bb3ed69065a5129a007e9aa50 | null | 921A:1353DF:3C80B25:479CEAD:6627D015 | null | MISS | cache-iad-kiad7000072-IAD | S1713885206.669710,VS0,VE14 | null | null | en | og:image | https://wasp-lang.dev/docs/tutorial/auth | og:url | 7. Adding Authentication | Wasp | og:title | Most modern apps need a way to create and authenticate users. Wasp makes this as easy as possible with its first-class auth support. | og:description | 7. Adding Authentication | Wasp | null | 7. Adding Authentication
Most modern apps need a way to create and authenticate users. Wasp makes this as easy as possible with its first-class auth support.
To add users to your app, you must:
Create a User Entity.
Tell Wasp to use the Username and Password authentication.
Add login and signup pages.
Update the main page to require authentication.
Add a relation between User and Task entities.
Modify your Queries and Actions so users can only see and modify their tasks.
Add a logout button.
Creating a User Entity
Since Wasp manages authentication, it will create the auth related entities for you in the background. Nothing to do here!
You must only add the User Entity to keep track of who owns which tasks.
main.wasp
// ...
entity User {=psl
id Int @id @default(autoincrement())
psl=}
Adding Auth to the Project
Next, tell Wasp to use full-stack authentication:
main.wasp
app TodoApp {
wasp: {
version: "^0.13.0"
},
title: "TodoApp",
auth: {
// Tells Wasp which entity to use for storing users.
userEntity: User,
methods: {
// Enable username and password auth.
usernameAndPassword: {}
},
// We'll see how this is used in a bit.
onAuthFailedRedirectTo: "/login"
}
}
// ...
Don't forget to update the database schema by running:
By doing this, Wasp will create:
Auth UI with login and signup forms.
A logout() action.
A React hook useAuth().
context.user for use in Queries and Actions.
info
Wasp also supports authentication using Google, GitHub, and email, with more on the way!
Adding Login and Signup Pages
Wasp creates the login and signup forms for us, but we still need to define the pages to display those forms on. We'll start by declaring the pages in the Wasp file:
JavaScript
TypeScript
main.wasp
// ...
route SignupRoute { path: "/signup", to: SignupPage }
page SignupPage {
component: import { SignupPage } from "@src/SignupPage"
}
route LoginRoute { path: "/login", to: LoginPage }
page LoginPage {
component: import { LoginPage } from "@src/LoginPage"
}
Great, Wasp now knows these pages exist!
Here's the React code for the pages you've just imported:
JavaScript
TypeScript
src/LoginPage.jsx
import { Link } from 'react-router-dom'
import { LoginForm } from 'wasp/client/auth'
export const LoginPage = () => {
return (
<div style={{ maxWidth: '400px', margin: '0 auto' }}>
<LoginForm />
<br />
<span>
I don't have an account yet (<Link to="/signup">go to signup</Link>).
</span>
</div>
)
}
The signup page is very similar to the login page:
JavaScript
TypeScript
src/SignupPage.jsx
import { Link } from 'react-router-dom'
import { SignupForm } from 'wasp/client/auth'
export const SignupPage = () => {
return (
<div style={{ maxWidth: '400px', margin: '0 auto' }}>
<SignupForm />
<br />
<span>
I already have an account (<Link to="/login">go to login</Link>).
</span>
</div>
)
}
Update the Main Page to Require Auth
We don't want users who are not logged in to access the main page, because they won't be able to create any tasks. So let's make the page private by requiring the user to be logged in:
main.wasp
// ...
page MainPage {
authRequired: true,
component: import { MainPage } from "@src/MainPage"
}
Now that auth is required for this page, unauthenticated users will be redirected to /login, as we specified with app.auth.onAuthFailedRedirectTo.
Additionally, when authRequired is true, the page's React component will be provided a user object as prop.
JavaScript
TypeScript
src/MainPage.jsx
export const MainPage = ({ user }) => {
// Do something with the user
// ...
}
Ok, time to test this out. Navigate to the main page (/) of the app. You'll get redirected to /login, where you'll be asked to authenticate.
Since we just added users, you don't have an account yet. Go to the signup page and create one. You'll be sent back to the main page where you will now be able to see the TODO list!
Let's check out what the database looks like. Start the Prisma Studio:
You'll notice that we now have a User entity in the database alongside the Task entity.
However, you will notice that if you try logging in as different users and creating some tasks, all users share the same tasks. That's because we haven't yet updated the queries and actions to have per-user tasks. Let's do that next.
You might notice some extra Prisma models like Auth, AuthIdentity and Session that Wasp created for us. You don't need to care about these right now, but if you are curious, you can read more about them here.
Defining a User-Task Relation
First, let's define a one-to-many relation between users and tasks (check the Prisma docs on relations):
main.wasp
// ...
entity User {=psl
id Int @id @default(autoincrement())
tasks Task[]
psl=}
entity Task {=psl
id Int @id @default(autoincrement())
description String
isDone Boolean @default(false)
user User? @relation(fields: [userId], references: [id])
userId Int?
psl=}
// ...
As always, you must migrate the database after changing the Entities:
note
We made user and userId in Task optional (via ?) because that allows us to keep the existing tasks, which don't have a user assigned, in the database.
This isn't recommended because it allows an unwanted state in the database (what is the purpose of the task not belonging to anybody?) and normally we would not make these fields optional.
Instead, we would do a data migration to take care of those tasks, even if it means just deleting them all. However, for this tutorial, for the sake of simplicity, we will stick with this.
Updating Operations to Check Authentication
Next, let's update the queries and actions to forbid access to non-authenticated users and to operate only on the currently logged-in user's tasks:
JavaScript
TypeScript
src/queries.js
import { HttpError } from 'wasp/server'
export const getTasks = async (args, context) => {
if (!context.user) {
throw new HttpError(401)
}
return context.entities.Task.findMany({
where: { user: { id: context.user.id } },
orderBy: { id: 'asc' },
})
}
JavaScript
TypeScript
src/actions.js
import { HttpError } from 'wasp/server'
export const createTask = async (args, context) => {
if (!context.user) {
throw new HttpError(401)
}
return context.entities.Task.create({
data: {
description: args.description,
user: { connect: { id: context.user.id } },
},
})
}
export const updateTask = async (args, context) => {
if (!context.user) {
throw new HttpError(401)
}
return context.entities.Task.updateMany({
where: { id: args.id, user: { id: context.user.id } },
data: { isDone: args.isDone },
})
}
note
Due to how Prisma works, we had to convert update to updateMany in updateTask action to be able to specify the user id in where.
With these changes, each user should have a list of tasks that only they can see and edit.
Try playing around, adding a few users and some tasks for each of them. Then open the DB studio:
You will see that each user has their tasks, just as we specified in our code!
Logout Button
Last, but not least, let's add the logout functionality:
JavaScript
TypeScript
src/MainPage.jsx
// ...
import { logout } from 'wasp/client/auth'
//...
const MainPage = () => {
// ...
return (
<div>
// ...
<button onClick={logout}>Logout</button>
</div>
)
}
This is it, we have a working authentication system, and our Todo app is multi-user!
What's Next?
We did it 🎉 You've followed along with this tutorial to create a basic Todo app with Wasp.
You should be ready to learn about more complicated features and go more in-depth with the features already covered. Scroll through the sidebar on the left side of the page to see every feature Wasp has to offer. Or, let your imagination run wild and start building your app! ✨
Looking for inspiration?
Get a jump start on your next project with Starter Templates
Make a real-time app with Web Sockets
note
If you notice that some of the features you'd like to have are missing, or have any other kind of feedback, please write to us on Discord or create an issue on Github, so we can learn which features to add/improve next 🙏
If you would like to contribute or help to build a feature, let us know! You can find more details on contributing here.
Oh, and do subscribe to our newsletter! We usually send one per month, and Matija does his best to unleash his creativity to make them engaging and fun to read :D! | https://wasp-lang.dev/docs/tutorial/auth |
|
1 | null | 2024-04-23T15:13:28.352Z | https://wasp-lang.dev/docs/data-model/entities | https://wasp-lang.dev/docs | http | ## Entities
Entities are the foundation of your app's data model. In short, an Entity defines a model in your database.
Wasp uses the excellent [Prisma ORM](https://www.prisma.io/) to implement all database functionality and occasionally enhances it with a thin abstraction layer. Wasp Entities directly correspond to [Prisma's data model](https://www.prisma.io/docs/concepts/components/prisma-schema/data-model). Still, you don't need to be familiar with Prisma to effectively use Wasp, as it comes with a simple API wrapper for working with Prisma's core features.
The only requirement for defining Wasp Entities is familiarity with the **_Prisma Schema Language (PSL)_**, a simple definition language explicitly created for defining models in Prisma. The language is declarative and very intuitive. We'll also go through an example later in the text, so there's no need to go and thoroughly learn it right away. Still, if you're curious, look no further than Prisma's official documentation:
* [Basic intro and examples](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-schema)
* [A more exhaustive language specification](https://www.prisma.io/docs/reference/api-reference/prisma-schema-reference)
## Defining an Entity[](#defining-an-entity "Direct link to Defining an Entity")
As mentioned, an `entity` declaration represents a database model.
Each `Entity` declaration corresponds 1-to-1 to [Prisma's data model](https://www.prisma.io/docs/concepts/components/prisma-schema/data-model). Here's how you could define an Entity that represents a Task:
* JavaScript
* TypeScript
```
entity Task {=psl id Int @id @default(autoincrement()) description String isDone Boolean @default(false)psl=}
```
Let's go through this declaration in detail:
* `entity Task` - This tells Wasp that we wish to define an Entity (i.e., database model) called `Task`. Wasp automatically creates a table called `tasks`.
* `{=psl ... psl=}` - Wasp treats everything that comes between the two `psl` tags as [PSL (Prisma Schema Language)](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-schema).
The above PSL definition tells Wasp to create a table for storing Tasks where each task has three fields (i.e., the `tasks` table has three columns):
* `id` - An integer value serving as a primary key. The database automatically generates it by incrementing the previously generated `id`.
* `description` - A string value for storing the task's description.
* `isDone` - A boolean value indicating the task's completion status. If you don't set it when creating a new task, the database sets it to `false` by default.
### Working with Entities[](#working-with-entities "Direct link to Working with Entities")
Let's see how you can define and work with Wasp Entities:
1. Create/update some Entities in your `.wasp` file.
2. Run `wasp db migrate-dev`. This command syncs the database model with the Entity definitions in your `.wasp` file. It does this by creating migration scripts.
3. Migration scripts are automatically placed in the `migrations/` folder. Make sure to commit this folder into version control.
4. Use Wasp's JavasScript API to work with the database when implementing Operations (we'll cover this in detail when we talk about [operations](https://wasp-lang.dev/docs/data-model/operations/overview)).
#### Using Entities in Operations[](#using-entities-in-operations "Direct link to Using Entities in Operations")
Most of the time, you will be working with Entities within the context of [Operations (Queries & Actions)](https://wasp-lang.dev/docs/data-model/operations/overview). We'll see how that's done on the next page.
#### Using Entities directly[](#using-entities-directly "Direct link to Using Entities directly")
If you need more control, you can directly interact with Entities by importing and using the [Prisma Client](https://www.prisma.io/docs/concepts/components/prisma-client/crud). We recommend sticking with conventional Wasp-provided mechanisms, only resorting to directly using the Prisma client only if you need a feature Wasp doesn't provide.
You can only use the Prisma Client in your Wasp server code. You can import it like this:
* JavaScript
* TypeScript
```
import { prisma } from 'wasp/server'prisma.task.create({ description: "Read the Entities doc", isDone: true // almost :)})
```
### Next steps[](#next-steps "Direct link to Next steps")
Now that we've seen how to define Entities that represent Wasp's core data model, we'll see how to make the most of them in other parts of Wasp. Keep reading to learn all about Wasp Operations! | null | https://wasp-lang.dev/docs/data-model/entities | Entities are the foundation of your app's data model. In short, an Entity defines a model in your database. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecc37cd9d381e-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:13:28 GMT | Tue, 23 Apr 2024 15:23:28 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=1a3jnShUIfjBgA6AriuEjZW4uX2b8Mn91rixz73BOrG5QPvGGC9rGymTcyVJ29t79RoHDdkPhoHlSpQf%2FENE9YGzQuy2AoCpw0oD28tFRZDVaTCW7Xj1vHSIjzvctck5"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | e0350f89105576556a4c5d4d57cd3c44ffa6d804 | null | 6F5E:16C4F4:39094EC:440E927:6627D017 | null | MISS | cache-iad-kiad7000168-IAD | S1713885208.314930,VS0,VE16 | null | null | en | og:image | https://wasp-lang.dev/docs/data-model/entities | og:url | Entities | Wasp | og:title | Entities are the foundation of your app's data model. In short, an Entity defines a model in your database. | og:description | Entities | Wasp | null | Entities
Entities are the foundation of your app's data model. In short, an Entity defines a model in your database.
Wasp uses the excellent Prisma ORM to implement all database functionality and occasionally enhances it with a thin abstraction layer. Wasp Entities directly correspond to Prisma's data model. Still, you don't need to be familiar with Prisma to effectively use Wasp, as it comes with a simple API wrapper for working with Prisma's core features.
The only requirement for defining Wasp Entities is familiarity with the Prisma Schema Language (PSL), a simple definition language explicitly created for defining models in Prisma. The language is declarative and very intuitive. We'll also go through an example later in the text, so there's no need to go and thoroughly learn it right away. Still, if you're curious, look no further than Prisma's official documentation:
Basic intro and examples
A more exhaustive language specification
Defining an Entity
As mentioned, an entity declaration represents a database model.
Each Entity declaration corresponds 1-to-1 to Prisma's data model. Here's how you could define an Entity that represents a Task:
JavaScript
TypeScript
entity Task {=psl
id Int @id @default(autoincrement())
description String
isDone Boolean @default(false)
psl=}
Let's go through this declaration in detail:
entity Task - This tells Wasp that we wish to define an Entity (i.e., database model) called Task. Wasp automatically creates a table called tasks.
{=psl ... psl=} - Wasp treats everything that comes between the two psl tags as PSL (Prisma Schema Language).
The above PSL definition tells Wasp to create a table for storing Tasks where each task has three fields (i.e., the tasks table has three columns):
id - An integer value serving as a primary key. The database automatically generates it by incrementing the previously generated id.
description - A string value for storing the task's description.
isDone - A boolean value indicating the task's completion status. If you don't set it when creating a new task, the database sets it to false by default.
Working with Entities
Let's see how you can define and work with Wasp Entities:
Create/update some Entities in your .wasp file.
Run wasp db migrate-dev. This command syncs the database model with the Entity definitions in your .wasp file. It does this by creating migration scripts.
Migration scripts are automatically placed in the migrations/ folder. Make sure to commit this folder into version control.
Use Wasp's JavasScript API to work with the database when implementing Operations (we'll cover this in detail when we talk about operations).
Using Entities in Operations
Most of the time, you will be working with Entities within the context of Operations (Queries & Actions). We'll see how that's done on the next page.
Using Entities directly
If you need more control, you can directly interact with Entities by importing and using the Prisma Client. We recommend sticking with conventional Wasp-provided mechanisms, only resorting to directly using the Prisma client only if you need a feature Wasp doesn't provide.
You can only use the Prisma Client in your Wasp server code. You can import it like this:
JavaScript
TypeScript
import { prisma } from 'wasp/server'
prisma.task.create({
description: "Read the Entities doc",
isDone: true // almost :)
})
Next steps
Now that we've seen how to define Entities that represent Wasp's core data model, we'll see how to make the most of them in other parts of Wasp. Keep reading to learn all about Wasp Operations! | https://wasp-lang.dev/docs/data-model/entities |
|
1 | null | 2024-04-23T15:13:30.203Z | https://wasp-lang.dev/docs/data-model/operations/overview | https://wasp-lang.dev/docs | http | Version: 0.13.0
## Overview
While Entities enable help you define your app's data model and relationships, Operations are all about working with this data.
There are two kinds of Operations: [Queries](https://wasp-lang.dev/docs/data-model/operations/queries) and [Actions](https://wasp-lang.dev/docs/data-model/operations/actions). As their names suggest, Queries are meant for reading data, and Actions are meant for changing it (either by updating existing entries or creating new ones).
Keep reading to find out all there is to know about Operations in Wasp. | null | https://wasp-lang.dev/docs/data-model/operations/overview | While Entities enable help you define your app's data model and relationships, Operations are all about working with this data. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecc4369a59c64-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:13:30 GMT | Tue, 23 Apr 2024 15:23:30 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=5moGcmGQaXwg4ij5yrJQlen8floyrMtLMh2MmYmCqIJGYUCxghKtHLJWciAmuOp6TKCfI5gRNDc2ma1d%2BrUfn8qINhBLbRub7jONMcG3QzAQg%2BkbccQFFHphJk%2FHRXNs"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 10b2759965718400507004399d671eb13b360e46 | null | 63A2:35D1BB:3B3494A:46510D6:6627D01A | null | MISS | cache-iad-kiad7000154-IAD | S1713885210.174960,VS0,VE15 | null | null | en | og:image | https://wasp-lang.dev/docs/data-model/operations/overview | og:url | Overview | Wasp | og:title | While Entities enable help you define your app's data model and relationships, Operations are all about working with this data. | og:description | Overview | Wasp | null | Version: 0.13.0
Overview
While Entities enable help you define your app's data model and relationships, Operations are all about working with this data.
There are two kinds of Operations: Queries and Actions. As their names suggest, Queries are meant for reading data, and Actions are meant for changing it (either by updating existing entries or creating new ones).
Keep reading to find out all there is to know about Operations in Wasp. | https://wasp-lang.dev/docs/data-model/operations/overview |
|
1 | null | 2024-04-23T15:13:32.156Z | https://wasp-lang.dev/docs/data-model/operations/queries | https://wasp-lang.dev/docs | http | ## Queries
We'll explain what Queries are and how to use them. If you're looking for a detailed API specification, skip ahead to the [API Reference](#api-reference).
You can use Queries to fetch data from the server. They shouldn't modify the server's state. Fetching all comments on a blog post, a list of users that liked a video, information about a single product based on its ID... All of these are perfect use cases for a Query.
tip
Queries are fairly similar to Actions in terms of their API. Therefore, if you're already familiar with Actions, you might find reading the entire guide repetitive.
We instead recommend skipping ahead and only reading [the differences between Queries and Actions](https://wasp-lang.dev/docs/data-model/operations/actions#differences-between-queries-and-actions), and consulting the [API Reference](#api-reference) as needed.
## Working with Queries[](#working-with-queries "Direct link to Working with Queries")
You declare queries in the `.wasp` file and implement them using NodeJS. Wasp not only runs these queries within the server's context but also creates code that enables you to call them from any part of your codebase, whether it's on the client or server side.
This means you don't have to build an HTTP API for your query, manage server-side request handling, or even deal with client-side response handling and caching. Instead, just concentrate on implementing the business logic inside your query, and let Wasp handle the rest!
To create a Query, you must:
1. Declare the Query in Wasp using the `query` declaration.
2. Define the Query's NodeJS implementation.
After completing these two steps, you'll be able to use the Query from any point in your code.
### Declaring Queries[](#declaring-queries "Direct link to Declaring Queries")
To create a Query in Wasp, we begin with a `query` declaration.
Let's declare two Queries - one to fetch all tasks, and another to fetch tasks based on a filter, such as whether a task is done:
* JavaScript
* TypeScript
main.wasp
```
// ...query getAllTasks { fn: import { getAllTasks } from "@src/queries.js"}query getFilteredTasks { fn: import { getFilteredTasks } from "@src/queries.js"}
```
If you want to know about all supported options for the `query` declaration, take a look at the [API Reference](#api-reference).
The names of Wasp Queries and their implementations don't need to match, but we'll keep them the same to avoid confusion.
info
You might have noticed that we told Wasp to import Query implementations that don't yet exist. Don't worry about that for now. We'll write the implementations imported from `queries.ts` in the next section.
It's a good idea to start with the high-level concept (i.e., the Query declaration in the Wasp file) and only then deal with the implementation details (i.e., the Query's implementation in JavaScript).
After declaring a Wasp Query, two important things happen:
* Wasp **generates a server-side NodeJS function** that shares its name with the Query.
* Wasp **generates a client-side JavaScript function** that shares its name with the Query (e.g., `getFilteredTasks`). This function takes a single optional argument - an object containing any serializable data you wish to use inside the Query. Wasp will send this object over the network and pass it into the Query's implementation as its first positional argument (more on this when we look at the implementations). Such an abstraction works thanks to an HTTP API route handler Wasp generates on the server, which calls the Query's NodeJS implementation under the hood.
Generating these two functions ensures a uniform calling interface across the entire app (both client and server).
### Implementing Queries in Node[](#implementing-queries-in-node "Direct link to Implementing Queries in Node")
Now that we've declared the Query, what remains is to implement it. We've instructed Wasp to look for the Queries' implementations in the file `src/queries.ts`, so that's where we should export them from.
Here's how you might implement the previously declared Queries `getAllTasks` and `getFilteredTasks`:
* JavaScript
* TypeScript
src/queries.js
```
// our "database"const tasks = [ { id: 1, description: 'Buy some eggs', isDone: true }, { id: 2, description: 'Make an omelette', isDone: false }, { id: 3, description: 'Eat breakfast', isDone: false },]// You don't need to use the arguments if you don't need themexport const getAllTasks = () => { return tasks}// The 'args' object is something sent by the caller (most often from the client)export const getFilteredTasks = (args) => { const { isDone } = args return tasks.filter((task) => task.isDone === isDone)}
```
tip
Wasp uses [superjson](https://github.com/blitz-js/superjson) under the hood. This means you're not limited to only sending and receiving JSON payloads.
You can send and receive any superjson-compatible payload (like Dates, Sets, Lists, circular references, etc.) and let Wasp handle the (de)serialization.
For a detailed explanation of the Query definition API (i.e., arguments and return values), check the [API Reference](#api-reference).
### Using Queries[](#using-queries "Direct link to Using Queries")
To use a Query, you can import it from `wasp/client/operations` and call it directly. As mentioned, the usage doesn't change depending on whether you're on the server or the client:
* JavaScript
* TypeScript
```
import { getAllTasks, getFilteredTasks } from 'wasp/client/operations'// ...const allTasks = await getAllTasks()const doneTasks = await getFilteredTasks({ isDone: true })
```
#### The `useQuery` hook[](#the-usequery-hook "Direct link to the-usequery-hook")
When using Queries on the client, you can make them reactive with the `useQuery` hook. This hook comes bundled with Wasp and is a thin wrapper around the `useQuery` hook from [_react-query_](https://github.com/tannerlinsley/react-query). The only difference is that you don't need to supply the key - Wasp handles this for you automatically.
Here's an example of calling the Queries using the `useQuery` hook:
* JavaScript
* TypeScript
src/MainPage.jsx
```
import React from 'react'import { useQuery, getAllTasks, getFilteredTasks } from 'wasp/client/operations'const MainPage = () => { const { data: allTasks, error: error1 } = useQuery(getAllTasks) const { data: doneTasks, error: error2 } = useQuery(getFilteredTasks, { isDone: true, }) if (error1 !== null || error2 !== null) { return <div>There was an error</div> } return ( <div> <h2>All Tasks</h2> {allTasks && allTasks.length > 0 ? allTasks.map((task) => <Task key={task.id} {...task} />) : 'No tasks'} <h2>Finished Tasks</h2> {doneTasks && doneTasks.length > 0 ? doneTasks.map((task) => <Task key={task.id} {...task} />) : 'No finished tasks'} </div> )}const Task = ({ description, isDone }: Task) => { return ( <div> <p> <strong>Description: </strong> {description} </p> <p> <strong>Is done: </strong> {isDone ? 'Yes' : 'No'} </p> </div> )}export default MainPage
```
For a detailed specification of the `useQuery` hook, check the [API Reference](#api-reference).
### Error Handling[](#error-handling "Direct link to Error Handling")
For security reasons, all exceptions thrown in the Query's NodeJS implementation are sent to the client as responses with the HTTP status code `500`, with all other details removed. Hiding error details by default helps against accidentally leaking possibly sensitive information over the network.
If you do want to pass additional error information to the client, you can construct and throw an appropriate `HttpError` in your implementation:
* JavaScript
* TypeScript
src/queries.js
```
import { HttpError } from 'wasp/server'export const getAllTasks = async (args, context) => { throw new HttpError( 403, // status code "You can't do this!", // message { foo: 'bar' } // data )}
```
If the status code is `4xx`, the client will receive a response object with the corresponding `message` and `data` fields, and it will rethrow the error (including these fields). To prevent information leakage, the server won't forward these fields for any other HTTP status codes.
### Using Entities in Queries[](#using-entities-in-queries "Direct link to Using Entities in Queries")
In most cases, resources used in Queries will be [Entities](https://wasp-lang.dev/docs/data-model/entities). To use an Entity in your Query, add it to the `query` declaration in Wasp:
* JavaScript
* TypeScript
main.wasp
```
query getAllTasks { fn: import { getAllTasks } from "@src/queries.js", entities: [Task]}query getFilteredTasks { fn: import { getFilteredTasks } from "@src/queries.js", entities: [Task]}
```
Wasp will inject the specified Entity into the Query's `context` argument, giving you access to the Entity's Prisma API:
* JavaScript
* TypeScript
src/queries.js
```
export const getAllTasks = async (args, context) => { return context.entities.Task.findMany({})}export const getFilteredTasks = async (args, context) => { return context.entities.Task.findMany({ where: { isDone: args.isDone }, })}
```
The object `context.entities.Task` exposes `prisma.task` from [Prisma's CRUD API](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-client/crud).
## API Reference[](#api-reference "Direct link to API Reference")
### Declaring Queries[](#declaring-queries-1 "Direct link to Declaring Queries")
The `query` declaration supports the following fields:
* `fn: ExtImport` required
The import statement of the Query's NodeJs implementation.
* `entities: [Entity]`
A list of entities you wish to use inside your Query. For instructions on using Entities in Queries, take a look at [the guide](#using-entities-in-queries).
#### Example[](#example "Direct link to Example")
* JavaScript
* TypeScript
Declaring the Query:
```
query getFoo { fn: import { getFoo } from "@src/queries.js" entities: [Foo]}
```
Enables you to import and use it anywhere in your code (on the server or the client):
```
import { getFoo } from 'wasp/client/operations'
```
### Implementing Queries[](#implementing-queries "Direct link to Implementing Queries")
The Query's implementation is a NodeJS function that takes two arguments (it can be an `async` function if you need to use the `await` keyword). Since both arguments are positional, you can name the parameters however you want, but we'll stick with `args` and `context`:
1. `args` (type depends on the Query)
An object containing the data **passed in when calling the query** (e.g., filtering conditions). Check [the usage examples](#using-queries) to see how to pass this object to the Query.
2. `context` (type depends on the Query)
An additional context object **passed into the Query by Wasp**. This object contains user session information, as well as information about entities. Check the [section about using entities in Queries](#using-entities-in-queries) to see how to use the entities field on the `context` object, or the [auth section](https://wasp-lang.dev/docs/auth/overview#using-the-contextuser-object) to see how to use the `user` object.
#### Example[](#example-1 "Direct link to Example")
* JavaScript
* TypeScript
The following Query:
```
query getFoo { fn: import { getFoo } from "@src/queries.js" entities: [Foo]}
```
Expects to find a named export `getFoo` from the file `src/queries.js`
queries.js
```
export const getFoo = (args, context) => { // implementation}
```
### The `useQuery` Hook[](#the-usequery-hook-1 "Direct link to the-usequery-hook-1")
Wasp's `useQuery` hook is a thin wrapper around the `useQuery` hook from [_react-query_](https://github.com/tannerlinsley/react-query). One key difference is that Wasp doesn't expect you to supply the cache key - it takes care of it under the hood.
Wasp's `useQuery` hook accepts three arguments:
* `queryFn` required
The client-side query function generated by Wasp based on a `query` declaration in your `.wasp` file.
* `queryFnArgs`
The arguments object (payload) you wish to pass into the Query. The Query's NodeJS implementation will receive this object as its first positional argument.
* `options`
A _react-query_ `options` object. Use this to change [the default behavior](https://react-query.tanstack.com/guides/important-defaults) for this particular Query. If you want to change the global defaults, you can do so in the [client setup function](https://wasp-lang.dev/docs/project/client-config#overriding-default-behaviour-for-queries).
For an example of usage, check [this section](#the-usequery-hook). | null | https://wasp-lang.dev/docs/data-model/operations/queries | We'll explain what Queries are and how to use them. If you're looking for a detailed API specification, skip ahead to the API Reference. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecc4f6c506fbf-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:13:32 GMT | Tue, 23 Apr 2024 15:23:32 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=sY3A4N6B5wTsial6t7O%2BIRnQ0RwbBRRfFopg3Ywgn1XhgXMGhjsOAbcAacg%2FBvG2yPIcXJM6foGf8eFGBK5xt%2Bi90p3hNuGY3e5nY3gF1gD1yfl6yqgmsF5uOLMdLl%2BJ"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 56c7e9eef9933158157c161d0a7136594ebf6885 | null | BA80:1D639D:3AF6585:45FB645:6627D01B | null | MISS | cache-iad-kiad7000022-IAD | S1713885212.084205,VS0,VE22 | null | null | en | og:image | https://wasp-lang.dev/docs/data-model/operations/queries | og:url | Queries | Wasp | og:title | We'll explain what Queries are and how to use them. If you're looking for a detailed API specification, skip ahead to the API Reference. | og:description | Queries | Wasp | null | Queries
We'll explain what Queries are and how to use them. If you're looking for a detailed API specification, skip ahead to the API Reference.
You can use Queries to fetch data from the server. They shouldn't modify the server's state. Fetching all comments on a blog post, a list of users that liked a video, information about a single product based on its ID... All of these are perfect use cases for a Query.
tip
Queries are fairly similar to Actions in terms of their API. Therefore, if you're already familiar with Actions, you might find reading the entire guide repetitive.
We instead recommend skipping ahead and only reading the differences between Queries and Actions, and consulting the API Reference as needed.
Working with Queries
You declare queries in the .wasp file and implement them using NodeJS. Wasp not only runs these queries within the server's context but also creates code that enables you to call them from any part of your codebase, whether it's on the client or server side.
This means you don't have to build an HTTP API for your query, manage server-side request handling, or even deal with client-side response handling and caching. Instead, just concentrate on implementing the business logic inside your query, and let Wasp handle the rest!
To create a Query, you must:
Declare the Query in Wasp using the query declaration.
Define the Query's NodeJS implementation.
After completing these two steps, you'll be able to use the Query from any point in your code.
Declaring Queries
To create a Query in Wasp, we begin with a query declaration.
Let's declare two Queries - one to fetch all tasks, and another to fetch tasks based on a filter, such as whether a task is done:
JavaScript
TypeScript
main.wasp
// ...
query getAllTasks {
fn: import { getAllTasks } from "@src/queries.js"
}
query getFilteredTasks {
fn: import { getFilteredTasks } from "@src/queries.js"
}
If you want to know about all supported options for the query declaration, take a look at the API Reference.
The names of Wasp Queries and their implementations don't need to match, but we'll keep them the same to avoid confusion.
info
You might have noticed that we told Wasp to import Query implementations that don't yet exist. Don't worry about that for now. We'll write the implementations imported from queries.ts in the next section.
It's a good idea to start with the high-level concept (i.e., the Query declaration in the Wasp file) and only then deal with the implementation details (i.e., the Query's implementation in JavaScript).
After declaring a Wasp Query, two important things happen:
Wasp generates a server-side NodeJS function that shares its name with the Query.
Wasp generates a client-side JavaScript function that shares its name with the Query (e.g., getFilteredTasks). This function takes a single optional argument - an object containing any serializable data you wish to use inside the Query. Wasp will send this object over the network and pass it into the Query's implementation as its first positional argument (more on this when we look at the implementations). Such an abstraction works thanks to an HTTP API route handler Wasp generates on the server, which calls the Query's NodeJS implementation under the hood.
Generating these two functions ensures a uniform calling interface across the entire app (both client and server).
Implementing Queries in Node
Now that we've declared the Query, what remains is to implement it. We've instructed Wasp to look for the Queries' implementations in the file src/queries.ts, so that's where we should export them from.
Here's how you might implement the previously declared Queries getAllTasks and getFilteredTasks:
JavaScript
TypeScript
src/queries.js
// our "database"
const tasks = [
{ id: 1, description: 'Buy some eggs', isDone: true },
{ id: 2, description: 'Make an omelette', isDone: false },
{ id: 3, description: 'Eat breakfast', isDone: false },
]
// You don't need to use the arguments if you don't need them
export const getAllTasks = () => {
return tasks
}
// The 'args' object is something sent by the caller (most often from the client)
export const getFilteredTasks = (args) => {
const { isDone } = args
return tasks.filter((task) => task.isDone === isDone)
}
tip
Wasp uses superjson under the hood. This means you're not limited to only sending and receiving JSON payloads.
You can send and receive any superjson-compatible payload (like Dates, Sets, Lists, circular references, etc.) and let Wasp handle the (de)serialization.
For a detailed explanation of the Query definition API (i.e., arguments and return values), check the API Reference.
Using Queries
To use a Query, you can import it from wasp/client/operations and call it directly. As mentioned, the usage doesn't change depending on whether you're on the server or the client:
JavaScript
TypeScript
import { getAllTasks, getFilteredTasks } from 'wasp/client/operations'
// ...
const allTasks = await getAllTasks()
const doneTasks = await getFilteredTasks({ isDone: true })
The useQuery hook
When using Queries on the client, you can make them reactive with the useQuery hook. This hook comes bundled with Wasp and is a thin wrapper around the useQuery hook from react-query. The only difference is that you don't need to supply the key - Wasp handles this for you automatically.
Here's an example of calling the Queries using the useQuery hook:
JavaScript
TypeScript
src/MainPage.jsx
import React from 'react'
import { useQuery, getAllTasks, getFilteredTasks } from 'wasp/client/operations'
const MainPage = () => {
const { data: allTasks, error: error1 } = useQuery(getAllTasks)
const { data: doneTasks, error: error2 } = useQuery(getFilteredTasks, {
isDone: true,
})
if (error1 !== null || error2 !== null) {
return <div>There was an error</div>
}
return (
<div>
<h2>All Tasks</h2>
{allTasks && allTasks.length > 0
? allTasks.map((task) => <Task key={task.id} {...task} />)
: 'No tasks'}
<h2>Finished Tasks</h2>
{doneTasks && doneTasks.length > 0
? doneTasks.map((task) => <Task key={task.id} {...task} />)
: 'No finished tasks'}
</div>
)
}
const Task = ({ description, isDone }: Task) => {
return (
<div>
<p>
<strong>Description: </strong>
{description}
</p>
<p>
<strong>Is done: </strong>
{isDone ? 'Yes' : 'No'}
</p>
</div>
)
}
export default MainPage
For a detailed specification of the useQuery hook, check the API Reference.
Error Handling
For security reasons, all exceptions thrown in the Query's NodeJS implementation are sent to the client as responses with the HTTP status code 500, with all other details removed. Hiding error details by default helps against accidentally leaking possibly sensitive information over the network.
If you do want to pass additional error information to the client, you can construct and throw an appropriate HttpError in your implementation:
JavaScript
TypeScript
src/queries.js
import { HttpError } from 'wasp/server'
export const getAllTasks = async (args, context) => {
throw new HttpError(
403, // status code
"You can't do this!", // message
{ foo: 'bar' } // data
)
}
If the status code is 4xx, the client will receive a response object with the corresponding message and data fields, and it will rethrow the error (including these fields). To prevent information leakage, the server won't forward these fields for any other HTTP status codes.
Using Entities in Queries
In most cases, resources used in Queries will be Entities. To use an Entity in your Query, add it to the query declaration in Wasp:
JavaScript
TypeScript
main.wasp
query getAllTasks {
fn: import { getAllTasks } from "@src/queries.js",
entities: [Task]
}
query getFilteredTasks {
fn: import { getFilteredTasks } from "@src/queries.js",
entities: [Task]
}
Wasp will inject the specified Entity into the Query's context argument, giving you access to the Entity's Prisma API:
JavaScript
TypeScript
src/queries.js
export const getAllTasks = async (args, context) => {
return context.entities.Task.findMany({})
}
export const getFilteredTasks = async (args, context) => {
return context.entities.Task.findMany({
where: { isDone: args.isDone },
})
}
The object context.entities.Task exposes prisma.task from Prisma's CRUD API.
API Reference
Declaring Queries
The query declaration supports the following fields:
fn: ExtImport required
The import statement of the Query's NodeJs implementation.
entities: [Entity]
A list of entities you wish to use inside your Query. For instructions on using Entities in Queries, take a look at the guide.
Example
JavaScript
TypeScript
Declaring the Query:
query getFoo {
fn: import { getFoo } from "@src/queries.js"
entities: [Foo]
}
Enables you to import and use it anywhere in your code (on the server or the client):
import { getFoo } from 'wasp/client/operations'
Implementing Queries
The Query's implementation is a NodeJS function that takes two arguments (it can be an async function if you need to use the await keyword). Since both arguments are positional, you can name the parameters however you want, but we'll stick with args and context:
args (type depends on the Query)
An object containing the data passed in when calling the query (e.g., filtering conditions). Check the usage examples to see how to pass this object to the Query.
context (type depends on the Query)
An additional context object passed into the Query by Wasp. This object contains user session information, as well as information about entities. Check the section about using entities in Queries to see how to use the entities field on the context object, or the auth section to see how to use the user object.
Example
JavaScript
TypeScript
The following Query:
query getFoo {
fn: import { getFoo } from "@src/queries.js"
entities: [Foo]
}
Expects to find a named export getFoo from the file src/queries.js
queries.js
export const getFoo = (args, context) => {
// implementation
}
The useQuery Hook
Wasp's useQuery hook is a thin wrapper around the useQuery hook from react-query. One key difference is that Wasp doesn't expect you to supply the cache key - it takes care of it under the hood.
Wasp's useQuery hook accepts three arguments:
queryFn required
The client-side query function generated by Wasp based on a query declaration in your .wasp file.
queryFnArgs
The arguments object (payload) you wish to pass into the Query. The Query's NodeJS implementation will receive this object as its first positional argument.
options
A react-query options object. Use this to change the default behavior for this particular Query. If you want to change the global defaults, you can do so in the client setup function.
For an example of usage, check this section. | https://wasp-lang.dev/docs/data-model/operations/queries |
|
1 | null | 2024-04-23T15:13:34.996Z | https://wasp-lang.dev/docs/data-model/operations/actions | https://wasp-lang.dev/docs | http | ## Actions
We'll explain what Actions are and how to use them. If you're looking for a detailed API specification, skip ahead to the [API Reference](#api-reference).
Actions are quite similar to [Queries](https://wasp-lang.dev/docs/data-model/operations/queries), but with a key distinction: Actions are designed to modify and add data, while Queries are solely for reading data. Examples of Actions include adding a comment to a blog post, liking a video, or updating a product's price.
Actions and Queries work together to keep data caches up-to-date.
tip
Actions are almost identical to Queries in terms of their API. Therefore, if you're already familiar with Queries, you might find reading the entire guide repetitive.
We instead recommend skipping ahead and only reading [the differences between Queries and Actions](#differences-between-queries-and-actions), and consulting the [API Reference](#api-reference) as needed.
## Working with Actions[](#working-with-actions "Direct link to Working with Actions")
Actions are declared in Wasp and implemented in NodeJS. Wasp runs Actions within the server's context, but it also generates code that allows you to call them from anywhere in your code (either client or server) using the same interface.
This means you don't have to worry about building an HTTP API for the Action, managing server-side request handling, or even dealing with client-side response handling and caching. Instead, just focus on developing the business logic inside your Action, and let Wasp handle the rest!
To create an Action, you need to:
1. Declare the Action in Wasp using the `action` declaration.
2. Implement the Action's NodeJS functionality.
Once these two steps are completed, you can use the Action from anywhere in your code.
### Declaring Actions[](#declaring-actions "Direct link to Declaring Actions")
To create an Action in Wasp, we begin with an `action` declaration. Let's declare two Actions - one for creating a task, and another for marking tasks as done:
* JavaScript
* TypeScript
main.wasp
```
// ...action createTask { fn: import { createTask } from "@src/actions.js"}action markTaskAsDone { fn: import { markTaskAsDone } from "@src/actions.js"}
```
If you want to know about all supported options for the `action` declaration, take a look at the [API Reference](#api-reference).
The names of Wasp Actions and their implementations don't necessarily have to match. However, to avoid confusion, we'll keep them the same.
tip
Wasp uses [superjson](https://github.com/blitz-js/superjson) under the hood. This means you're not limited to only sending and receiving JSON payloads.
You can send and receive any superjson-compatible payload (like Dates, Sets, Lists, circular references, etc.) and let Wasp handle the (de)serialization.
After declaring a Wasp Action, two important things happen:
* Wasp **generates a server-side NodeJS function** that shares its name with the Action.
* Wasp **generates a client-side JavaScript function** that shares its name with the Action (e.g., `markTaskAsDone`). This function takes a single optional argument - an object containing any serializable data you wish to use inside the Action. Wasp will send this object over the network and pass it into the Action's implementation as its first positional argument (more on this when we look at the implementations). Such an abstraction works thanks to an HTTP API route handler Wasp generates on the server, which calls the Action's NodeJS implementation under the hood.
Generating these two functions ensures a uniform calling interface across the entire app (both client and server).
### Implementing Actions in Node[](#implementing-actions-in-node "Direct link to Implementing Actions in Node")
Now that we've declared the Action, what remains is to implement it. We've instructed Wasp to look for the Actions' implementations in the file `src/actions.ts`, so that's where we should export them from.
Here's how you might implement the previously declared Actions `createTask` and `markTaskAsDone`:
* JavaScript
* TypeScript
src/actions.js
```
// our "database"let nextId = 4const tasks = [ { id: 1, description: 'Buy some eggs', isDone: true }, { id: 2, description: 'Make an omelette', isDone: false }, { id: 3, description: 'Eat breakfast', isDone: false },]// You don't need to use the arguments if you don't need themexport const createTask = (args) => { const newTask = { id: nextId, isDone: false, description: args.description, } nextId += 1 tasks.push(newTask) return newTask}// The 'args' object is something sent by the caller (most often from the client)export const markTaskAsDone = (args) => { const task = tasks.find((task) => task.id === args.id) if (!task) { // We'll show how to properly handle such errors later return } task.isDone = true}
```
tip
Wasp uses [superjson](https://github.com/blitz-js/superjson) under the hood. This means you're not limited to only sending and receiving JSON payloads.
You can send and receive any superjson-compatible payload (like Dates, Sets, Lists, circular references, etc.) and let Wasp handle the (de)serialization.
For a detailed explanation of the Action definition API (i.e., arguments and return values), check the [API Reference](#api-reference).
### Using Actions[](#using-actions "Direct link to Using Actions")
To use an Action, you can import it from `wasp/client/operations` and call it directly. As mentioned, the usage doesn't change depending on whether you're on the server or the client:
* JavaScript
* TypeScript
```
import { createTask, markTasAsDone } from 'wasp/client/operations'// ...const newTask = await createTask({ description: 'Learn TypeScript' })await markTasAsDone({ id: 1 })
```
When using Actions on the client, you'll most likely want to use them inside a component:
* JavaScript
* TypeScript
src/pages/Task.jsx
```
import React from 'react'import { useQuery, getTask, markTaskAsDone } from 'wasp/client/operations'export const TaskPage = ({ id }) => { const { data: task } = useQuery(getTask, { id }) if (!task) { return <h1>"Loading"</h1> } const { description, isDone } = task return ( <div> <p> <strong>Description: </strong> {description} </p> <p> <strong>Is done: </strong> {isDone ? 'Yes' : 'No'} </p> {isDone || ( <button onClick={() => markTaskAsDone({ id })}>Mark as done.</button> )} </div> )}
```
Since Actions don't require reactivity, they are safe to use inside components without a hook. Still, Wasp provides comes with the `useAction` hook you can use to enhance actions. Read all about it in the [API Reference](#api-reference).
### Error Handling[](#error-handling "Direct link to Error Handling")
For security reasons, all exceptions thrown in the Action's NodeJS implementation are sent to the client as responses with the HTTP status code `500`, with all other details removed. Hiding error details by default helps against accidentally leaking possibly sensitive information over the network.
If you do want to pass additional error information to the client, you can construct and throw an appropriate `HttpError` in your implementation:
* JavaScript
* TypeScript
src/actions.js
```
import { HttpError } from 'wasp/server'export const createTask = async (args, context) => { throw new HttpError( 403, // status code "You can't do this!", // message { foo: 'bar' } // data )}
```
### Using Entities in Actions[](#using-entities-in-actions "Direct link to Using Entities in Actions")
In most cases, resources used in Actions will be [Entities](https://wasp-lang.dev/docs/data-model/entities). To use an Entity in your Action, add it to the `action` declaration in Wasp:
* JavaScript
* TypeScript
main.wasp
```
action createTask { fn: import { createTask } from "@src/actions.js", entities: [Task]}action markTaskAsDone { fn: import { markTaskAsDone } from "@src/actions.js", entities: [Task]}
```
Wasp will inject the specified Entity into the Action's `context` argument, giving you access to the Entity's Prisma API. Wasp invalidates frontend Query caches by looking at the Entities used by each Action/Query. Read more about Wasp's smart cache invalidation [here](#cache-invalidation).
* JavaScript
* TypeScript
src/actions.js
```
// The 'args' object is the payload sent by the caller (most often from the client)export const createTask = async (args, context) => { const newTask = await context.entities.Task.create({ data: { description: args.description, isDone: false, }, }) return newTask}export const markTaskAsDone = async (args, context) => { await context.entities.Task.update({ where: { id: args.id }, data: { isDone: true }, })}
```
The object `context.entities.Task` exposes `prisma.task` from [Prisma's CRUD API](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-client/crud).
## Cache Invalidation[](#cache-invalidation "Direct link to Cache Invalidation")
One of the trickiest parts of managing a web app's state is making sure the data returned by the Queries is up to date. Since Wasp uses _react-query_ for Query management, we must make sure to invalidate Queries (more specifically, their cached results managed by _react-query_) whenever they become stale.
It's possible to invalidate the caches manually through several mechanisms _react-query_ provides (e.g., refetch, direct invalidation). However, since manual cache invalidation quickly becomes complex and error-prone, Wasp offers a faster and a more effective solution to get you started: **automatic Entity-based Query cache invalidation**. Because Actions can (and most often do) modify the state while Queries read it, Wasp invalidates a Query's cache whenever an Action that uses the same Entity is executed.
For example, if the Action `createTask` and Query `getTasks` both use the Entity `Task`, executing `createTask` may cause the cached result of `getTasks` to become outdated. In response, Wasp will invalidate it, causing `getTasks` to refetch data from the server and update it.
In practice, this means that Wasp keeps the Queries "fresh" without requiring you to think about cache invalidation.
On the other hand, this kind of automatic cache invalidation can become wasteful (some updates might not be necessary) and will only work for Entities. If that's an issue, you can use the mechanisms provided by _react-query_ for now, and expect more direct support in Wasp for handling those use cases in a nice, elegant way.
If you wish to optimistically set cache values after performing an Action, you can do so using [optimistic updates](https://stackoverflow.com/a/33009713). Configure them using Wasp's [useAction hook](#the-useaction-hook-and-optimistic-updates). This is currently the only manual cache invalidation mechanism Wasps supports natively. For everything else, you can always rely on _react-query_.
## Differences Between Queries and Actions[](#differences-between-queries-and-actions "Direct link to Differences Between Queries and Actions")
Actions and Queries are two closely related concepts in Wasp. They might seem to perform similar tasks, but Wasp treats them differently, and each concept represents a different thing.
Here are the key differences between Queries and Actions:
1. Actions can (and often should) modify the server's state, while Queries are only permitted to read it. Wasp relies on you adhering to this convention when performing cache invalidations, so it's crucial to follow it.
2. Actions don't need to be reactive, so you can call them directly. However, Wasp does provide a [`useAction` React hook](#the-useaction-hook-and-optimistic-updates) for adding extra behavior to the Action (like optimistic updates).
3. `action` declarations in Wasp are mostly identical to `query` declarations. The only difference lies in the declaration's name.
## API Reference[](#api-reference "Direct link to API Reference")
### Declaring Actions in Wasp[](#declaring-actions-in-wasp "Direct link to Declaring Actions in Wasp")
The `action` declaration supports the following fields:
* `fn: ExtImport` required
The import statement of the Action's NodeJs implementation.
* `entities: [Entity]`
A list of entities you wish to use inside your Action. For instructions on using Entities in Actions, take a look at [the guide](#using-entities-in-actions).
#### Example[](#example "Direct link to Example")
* JavaScript
* TypeScript
Declaring the Action:
```
query createFoo { fn: import { createFoo } from "@src/actions.js" entities: [Foo]}
```
Enables you to import and use it anywhere in your code (on the server or the client):
```
import { createFoo } from 'wasp/client/operations'
```
### Implementing Actions[](#implementing-actions "Direct link to Implementing Actions")
The Action's implementation is a NodeJS function that takes two arguments (it can be an `async` function if you need to use the `await` keyword). Since both arguments are positional, you can name the parameters however you want, but we'll stick with `args` and `context`:
1. `args` (type depends on the Action)
An object containing the data **passed in when calling the Action** (e.g., filtering conditions). Check [the usage examples](#using-actions) to see how to pass this object to the Action.
2. `context` (type depends on the Action)
An additional context object **passed into the Action by Wasp**. This object contains user session information, as well as information about entities. Check the [section about using entities in Actions](#using-entities-in-actions) to see how to use the entities field on the `context` object, or the [auth section](https://wasp-lang.dev/docs/auth/overview#using-the-contextuser-object) to see how to use the `user` object.
#### Example[](#example-1 "Direct link to Example")
* JavaScript
* TypeScript
The following Action:
```
action createFoo { fn: import { createFoo } from "@src/actions.js" entities: [Foo]}
```
Expects to find a named export `createfoo` from the file `src/actions.js`
actions.js
```
export const createFoo = (args, context) => { // implementation}
```
### The `useAction` Hook and Optimistic Updates[](#the-useaction-hook-and-optimistic-updates "Direct link to the-useaction-hook-and-optimistic-updates")
Make sure you understand how [Queries](https://wasp-lang.dev/docs/data-model/operations/queries) and [Cache Invalidation](#cache-invalidation) work before reading this chapter.
When using Actions in components, you can enhance them with the help of the `useAction` hook. This hook comes bundled with Wasp, and is used for decorating Wasp Actions. In other words, the hook returns a function whose API matches the original Action while also doing something extra under the hood (depending on how you configure it).
The `useAction` hook accepts two arguments:
* `actionFn` required
The Wasp Action (i.e., the client-side Action function generated by Wasp based on a Action declaration) you wish to enhance.
* `actionOptions`
An object configuring the extra features you want to add to the given Action. While this argument is technically optional, there is no point in using the `useAction` hook without providing it (it would be the same as using the Action directly). The Action options object supports the following fields:
* `optimisticUpdates`
An array of objects where each object defines an [optimistic update](https://stackoverflow.com/a/33009713) to perform on the Query cache. To define an optimistic update, you must specify the following properties:
* `getQuerySpecifier` required
A function returning the Query specifier (i.e., a value used to address the Query you want to update). A Query specifier is an array specifying the query function and arguments. For example, to optimistically update the Query used with `useQuery(fetchFilteredTasks, {isDone: true }]`, your `getQuerySpecifier` function would have to return the array `[fetchFilteredTasks, { isDone: true}]`. Wasp will forward the argument you pass into the decorated Action to this function (i.e., you can use the properties of the added/changed item to address the Query).
* `updateQuery` required
The function used to perform the optimistic update. It should return the desired state of the cache. Wasp will call it with the following arguments:
* `item` - The argument you pass into the decorated Action.
* `oldData` - The currently cached value for the Query identified by the specifier.
caution
The `updateQuery` function must be a pure function. It must return the desired cache value identified by the `getQuerySpecifier` function and _must not_ perform any side effects.
Also, make sure you only update the Query caches affected by your Action causing the optimistic update (Wasp cannot yet verify this).
Finally, your implementation of the `updateQuery` function should work correctly regardless of the state of `oldData` (e.g., don't rely on array positioning). If you need to do something else during your optimistic update, you can directly use _react-query_'s lower-level API (read more about it [here](#advanced-usage)).
Here's an example showing how to configure the Action `markTaskAsDone` that toggles a task's `isDone` status to perform an optimistic update:
* JavaScript
* TypeScript
src/pages/Task.jsx
```
import React from 'react'import { useQuery, useAction, getTask, markTaskAsDone,} from 'wasp/client/operations'const TaskPage = ({ id }) => { const { data: task } = useQuery(getTask, { id }) const markTaskAsDoneOptimistically = useAction(markTaskAsDone, { optimisticUpdates: [ { getQuerySpecifier: ({ id }) => [getTask, { id }], updateQuery: (_payload, oldData) => ({ ...oldData, isDone: true }), }, ], }) if (!task) { return <h1>"Loading"</h1> } const { description, isDone } = task return ( <div> <p> <strong>Description: </strong> {description} </p> <p> <strong>Is done: </strong> {isDone ? 'Yes' : 'No'} </p> {isDone || ( <button onClick={() => markTaskAsDoneOptimistically({ id })}> Mark as done. </button> )} </div> )}export default TaskPage
```
#### Advanced usage[](#advanced-usage "Direct link to Advanced usage")
The `useAction` hook currently only supports specifying optimistic updates. You can expect more features in future versions of Wasp.
Wasp's optimistic update API is deliberately small and focuses exclusively on updating Query caches (as that's the most common use case). You might need an API that offers more options or a higher level of control. If that's the case, instead of using Wasp's `useAction` hook, you can use _react-query_'s `useMutation` hook and directly work with [their low-level API](https://tanstack.com/query/v4/docs/guides/optimistic-updates?from=reactQueryV3&original=https://react-query-v3.tanstack.com/guides/optimistic-updates).
If you decide to use _react-query_'s API directly, you will need access to Query cache key. Wasp internally uses this key but abstracts it from the programmer. Still, you can easily obtain it by accessing the `queryCacheKey` property on any Query:
* JavaScript
* TypeScript
```
import { getTasks } from 'wasp/client/operations'const queryKey = getTasks.queryCacheKey
``` | null | https://wasp-lang.dev/docs/data-model/operations/actions | We'll explain what Actions are and how to use them. If you're looking for a detailed API specification, skip ahead to the API Reference. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecc612ea21fe3-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:13:34 GMT | Tue, 23 Apr 2024 15:23:34 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=MwABETvOkWiWPLZHD1PsIe%2FCgjfxbZhuIaWG%2BOw3NJkuLvji7pKZCjj0CcuXDAY6tWZ7BvUF3x9HOpBDtA2CmpTe%2BlXTm4u46Ji5e7%2FIFlm%2FhkgwJtIPFvuaRYr1t2W5"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 14bbf138af3b3ca7341cfc0f015adbcf7776bf7f | null | 93B4:1FE0FF:3C069EC:470BA47:6627D01D | HIT | MISS | cache-iad-kiad7000064-IAD | S1713885215.932191,VS0,VE11 | null | null | en | og:image | https://wasp-lang.dev/docs/data-model/operations/actions | og:url | Actions | Wasp | og:title | We'll explain what Actions are and how to use them. If you're looking for a detailed API specification, skip ahead to the API Reference. | og:description | Actions | Wasp | null | Actions
We'll explain what Actions are and how to use them. If you're looking for a detailed API specification, skip ahead to the API Reference.
Actions are quite similar to Queries, but with a key distinction: Actions are designed to modify and add data, while Queries are solely for reading data. Examples of Actions include adding a comment to a blog post, liking a video, or updating a product's price.
Actions and Queries work together to keep data caches up-to-date.
tip
Actions are almost identical to Queries in terms of their API. Therefore, if you're already familiar with Queries, you might find reading the entire guide repetitive.
We instead recommend skipping ahead and only reading the differences between Queries and Actions, and consulting the API Reference as needed.
Working with Actions
Actions are declared in Wasp and implemented in NodeJS. Wasp runs Actions within the server's context, but it also generates code that allows you to call them from anywhere in your code (either client or server) using the same interface.
This means you don't have to worry about building an HTTP API for the Action, managing server-side request handling, or even dealing with client-side response handling and caching. Instead, just focus on developing the business logic inside your Action, and let Wasp handle the rest!
To create an Action, you need to:
Declare the Action in Wasp using the action declaration.
Implement the Action's NodeJS functionality.
Once these two steps are completed, you can use the Action from anywhere in your code.
Declaring Actions
To create an Action in Wasp, we begin with an action declaration. Let's declare two Actions - one for creating a task, and another for marking tasks as done:
JavaScript
TypeScript
main.wasp
// ...
action createTask {
fn: import { createTask } from "@src/actions.js"
}
action markTaskAsDone {
fn: import { markTaskAsDone } from "@src/actions.js"
}
If you want to know about all supported options for the action declaration, take a look at the API Reference.
The names of Wasp Actions and their implementations don't necessarily have to match. However, to avoid confusion, we'll keep them the same.
tip
Wasp uses superjson under the hood. This means you're not limited to only sending and receiving JSON payloads.
You can send and receive any superjson-compatible payload (like Dates, Sets, Lists, circular references, etc.) and let Wasp handle the (de)serialization.
After declaring a Wasp Action, two important things happen:
Wasp generates a server-side NodeJS function that shares its name with the Action.
Wasp generates a client-side JavaScript function that shares its name with the Action (e.g., markTaskAsDone). This function takes a single optional argument - an object containing any serializable data you wish to use inside the Action. Wasp will send this object over the network and pass it into the Action's implementation as its first positional argument (more on this when we look at the implementations). Such an abstraction works thanks to an HTTP API route handler Wasp generates on the server, which calls the Action's NodeJS implementation under the hood.
Generating these two functions ensures a uniform calling interface across the entire app (both client and server).
Implementing Actions in Node
Now that we've declared the Action, what remains is to implement it. We've instructed Wasp to look for the Actions' implementations in the file src/actions.ts, so that's where we should export them from.
Here's how you might implement the previously declared Actions createTask and markTaskAsDone:
JavaScript
TypeScript
src/actions.js
// our "database"
let nextId = 4
const tasks = [
{ id: 1, description: 'Buy some eggs', isDone: true },
{ id: 2, description: 'Make an omelette', isDone: false },
{ id: 3, description: 'Eat breakfast', isDone: false },
]
// You don't need to use the arguments if you don't need them
export const createTask = (args) => {
const newTask = {
id: nextId,
isDone: false,
description: args.description,
}
nextId += 1
tasks.push(newTask)
return newTask
}
// The 'args' object is something sent by the caller (most often from the client)
export const markTaskAsDone = (args) => {
const task = tasks.find((task) => task.id === args.id)
if (!task) {
// We'll show how to properly handle such errors later
return
}
task.isDone = true
}
tip
Wasp uses superjson under the hood. This means you're not limited to only sending and receiving JSON payloads.
You can send and receive any superjson-compatible payload (like Dates, Sets, Lists, circular references, etc.) and let Wasp handle the (de)serialization.
For a detailed explanation of the Action definition API (i.e., arguments and return values), check the API Reference.
Using Actions
To use an Action, you can import it from wasp/client/operations and call it directly. As mentioned, the usage doesn't change depending on whether you're on the server or the client:
JavaScript
TypeScript
import { createTask, markTasAsDone } from 'wasp/client/operations'
// ...
const newTask = await createTask({ description: 'Learn TypeScript' })
await markTasAsDone({ id: 1 })
When using Actions on the client, you'll most likely want to use them inside a component:
JavaScript
TypeScript
src/pages/Task.jsx
import React from 'react'
import { useQuery, getTask, markTaskAsDone } from 'wasp/client/operations'
export const TaskPage = ({ id }) => {
const { data: task } = useQuery(getTask, { id })
if (!task) {
return <h1>"Loading"</h1>
}
const { description, isDone } = task
return (
<div>
<p>
<strong>Description: </strong>
{description}
</p>
<p>
<strong>Is done: </strong>
{isDone ? 'Yes' : 'No'}
</p>
{isDone || (
<button onClick={() => markTaskAsDone({ id })}>Mark as done.</button>
)}
</div>
)
}
Since Actions don't require reactivity, they are safe to use inside components without a hook. Still, Wasp provides comes with the useAction hook you can use to enhance actions. Read all about it in the API Reference.
Error Handling
For security reasons, all exceptions thrown in the Action's NodeJS implementation are sent to the client as responses with the HTTP status code 500, with all other details removed. Hiding error details by default helps against accidentally leaking possibly sensitive information over the network.
If you do want to pass additional error information to the client, you can construct and throw an appropriate HttpError in your implementation:
JavaScript
TypeScript
src/actions.js
import { HttpError } from 'wasp/server'
export const createTask = async (args, context) => {
throw new HttpError(
403, // status code
"You can't do this!", // message
{ foo: 'bar' } // data
)
}
Using Entities in Actions
In most cases, resources used in Actions will be Entities. To use an Entity in your Action, add it to the action declaration in Wasp:
JavaScript
TypeScript
main.wasp
action createTask {
fn: import { createTask } from "@src/actions.js",
entities: [Task]
}
action markTaskAsDone {
fn: import { markTaskAsDone } from "@src/actions.js",
entities: [Task]
}
Wasp will inject the specified Entity into the Action's context argument, giving you access to the Entity's Prisma API. Wasp invalidates frontend Query caches by looking at the Entities used by each Action/Query. Read more about Wasp's smart cache invalidation here.
JavaScript
TypeScript
src/actions.js
// The 'args' object is the payload sent by the caller (most often from the client)
export const createTask = async (args, context) => {
const newTask = await context.entities.Task.create({
data: {
description: args.description,
isDone: false,
},
})
return newTask
}
export const markTaskAsDone = async (args, context) => {
await context.entities.Task.update({
where: { id: args.id },
data: { isDone: true },
})
}
The object context.entities.Task exposes prisma.task from Prisma's CRUD API.
Cache Invalidation
One of the trickiest parts of managing a web app's state is making sure the data returned by the Queries is up to date. Since Wasp uses react-query for Query management, we must make sure to invalidate Queries (more specifically, their cached results managed by react-query) whenever they become stale.
It's possible to invalidate the caches manually through several mechanisms react-query provides (e.g., refetch, direct invalidation). However, since manual cache invalidation quickly becomes complex and error-prone, Wasp offers a faster and a more effective solution to get you started: automatic Entity-based Query cache invalidation. Because Actions can (and most often do) modify the state while Queries read it, Wasp invalidates a Query's cache whenever an Action that uses the same Entity is executed.
For example, if the Action createTask and Query getTasks both use the Entity Task, executing createTask may cause the cached result of getTasks to become outdated. In response, Wasp will invalidate it, causing getTasks to refetch data from the server and update it.
In practice, this means that Wasp keeps the Queries "fresh" without requiring you to think about cache invalidation.
On the other hand, this kind of automatic cache invalidation can become wasteful (some updates might not be necessary) and will only work for Entities. If that's an issue, you can use the mechanisms provided by react-query for now, and expect more direct support in Wasp for handling those use cases in a nice, elegant way.
If you wish to optimistically set cache values after performing an Action, you can do so using optimistic updates. Configure them using Wasp's useAction hook. This is currently the only manual cache invalidation mechanism Wasps supports natively. For everything else, you can always rely on react-query.
Differences Between Queries and Actions
Actions and Queries are two closely related concepts in Wasp. They might seem to perform similar tasks, but Wasp treats them differently, and each concept represents a different thing.
Here are the key differences between Queries and Actions:
Actions can (and often should) modify the server's state, while Queries are only permitted to read it. Wasp relies on you adhering to this convention when performing cache invalidations, so it's crucial to follow it.
Actions don't need to be reactive, so you can call them directly. However, Wasp does provide a useAction React hook for adding extra behavior to the Action (like optimistic updates).
action declarations in Wasp are mostly identical to query declarations. The only difference lies in the declaration's name.
API Reference
Declaring Actions in Wasp
The action declaration supports the following fields:
fn: ExtImport required
The import statement of the Action's NodeJs implementation.
entities: [Entity]
A list of entities you wish to use inside your Action. For instructions on using Entities in Actions, take a look at the guide.
Example
JavaScript
TypeScript
Declaring the Action:
query createFoo {
fn: import { createFoo } from "@src/actions.js"
entities: [Foo]
}
Enables you to import and use it anywhere in your code (on the server or the client):
import { createFoo } from 'wasp/client/operations'
Implementing Actions
The Action's implementation is a NodeJS function that takes two arguments (it can be an async function if you need to use the await keyword). Since both arguments are positional, you can name the parameters however you want, but we'll stick with args and context:
args (type depends on the Action)
An object containing the data passed in when calling the Action (e.g., filtering conditions). Check the usage examples to see how to pass this object to the Action.
context (type depends on the Action)
An additional context object passed into the Action by Wasp. This object contains user session information, as well as information about entities. Check the section about using entities in Actions to see how to use the entities field on the context object, or the auth section to see how to use the user object.
Example
JavaScript
TypeScript
The following Action:
action createFoo {
fn: import { createFoo } from "@src/actions.js"
entities: [Foo]
}
Expects to find a named export createfoo from the file src/actions.js
actions.js
export const createFoo = (args, context) => {
// implementation
}
The useAction Hook and Optimistic Updates
Make sure you understand how Queries and Cache Invalidation work before reading this chapter.
When using Actions in components, you can enhance them with the help of the useAction hook. This hook comes bundled with Wasp, and is used for decorating Wasp Actions. In other words, the hook returns a function whose API matches the original Action while also doing something extra under the hood (depending on how you configure it).
The useAction hook accepts two arguments:
actionFn required
The Wasp Action (i.e., the client-side Action function generated by Wasp based on a Action declaration) you wish to enhance.
actionOptions
An object configuring the extra features you want to add to the given Action. While this argument is technically optional, there is no point in using the useAction hook without providing it (it would be the same as using the Action directly). The Action options object supports the following fields:
optimisticUpdates
An array of objects where each object defines an optimistic update to perform on the Query cache. To define an optimistic update, you must specify the following properties:
getQuerySpecifier required
A function returning the Query specifier (i.e., a value used to address the Query you want to update). A Query specifier is an array specifying the query function and arguments. For example, to optimistically update the Query used with useQuery(fetchFilteredTasks, {isDone: true }], your getQuerySpecifier function would have to return the array [fetchFilteredTasks, { isDone: true}]. Wasp will forward the argument you pass into the decorated Action to this function (i.e., you can use the properties of the added/changed item to address the Query).
updateQuery required
The function used to perform the optimistic update. It should return the desired state of the cache. Wasp will call it with the following arguments:
item - The argument you pass into the decorated Action.
oldData - The currently cached value for the Query identified by the specifier.
caution
The updateQuery function must be a pure function. It must return the desired cache value identified by the getQuerySpecifier function and must not perform any side effects.
Also, make sure you only update the Query caches affected by your Action causing the optimistic update (Wasp cannot yet verify this).
Finally, your implementation of the updateQuery function should work correctly regardless of the state of oldData (e.g., don't rely on array positioning). If you need to do something else during your optimistic update, you can directly use react-query's lower-level API (read more about it here).
Here's an example showing how to configure the Action markTaskAsDone that toggles a task's isDone status to perform an optimistic update:
JavaScript
TypeScript
src/pages/Task.jsx
import React from 'react'
import {
useQuery,
useAction,
getTask,
markTaskAsDone,
} from 'wasp/client/operations'
const TaskPage = ({ id }) => {
const { data: task } = useQuery(getTask, { id })
const markTaskAsDoneOptimistically = useAction(markTaskAsDone, {
optimisticUpdates: [
{
getQuerySpecifier: ({ id }) => [getTask, { id }],
updateQuery: (_payload, oldData) => ({ ...oldData, isDone: true }),
},
],
})
if (!task) {
return <h1>"Loading"</h1>
}
const { description, isDone } = task
return (
<div>
<p>
<strong>Description: </strong>
{description}
</p>
<p>
<strong>Is done: </strong>
{isDone ? 'Yes' : 'No'}
</p>
{isDone || (
<button onClick={() => markTaskAsDoneOptimistically({ id })}>
Mark as done.
</button>
)}
</div>
)
}
export default TaskPage
Advanced usage
The useAction hook currently only supports specifying optimistic updates. You can expect more features in future versions of Wasp.
Wasp's optimistic update API is deliberately small and focuses exclusively on updating Query caches (as that's the most common use case). You might need an API that offers more options or a higher level of control. If that's the case, instead of using Wasp's useAction hook, you can use react-query's useMutation hook and directly work with their low-level API.
If you decide to use react-query's API directly, you will need access to Query cache key. Wasp internally uses this key but abstracts it from the programmer. Still, you can easily obtain it by accessing the queryCacheKey property on any Query:
JavaScript
TypeScript
import { getTasks } from 'wasp/client/operations'
const queryKey = getTasks.queryCacheKey | https://wasp-lang.dev/docs/data-model/operations/actions |
|
1 | null | 2024-04-23T15:13:37.700Z | https://wasp-lang.dev/docs/data-model/crud | https://wasp-lang.dev/docs | http | ## Automatic CRUD
If you have a lot of experience writing full-stack apps, you probably ended up doing some of the same things many times: listing data, adding data, editing it, and deleting it.
Wasp makes handling these boring bits easy by offering a higher-level concept called Automatic CRUD.
With a single declaration, you can tell Wasp to automatically generate server-side logic (i.e., Queries and Actions) for creating, reading, updating and deleting [Entities](https://wasp-lang.dev/docs/data-model/entities). As you update definitions for your Entities, Wasp automatically regenerates the backend logic.
Early preview
This feature is currently in early preview and we are actively working on it. Read more about [our plans](#future-of-crud-operations-in-wasp) for CRUD operations.
## Overview[](#overview "Direct link to Overview")
Imagine we have a `Task` entity and we want to enable CRUD operations for it.
main.wasp
```
entity Task {=psl id Int @id @default(autoincrement()) description String isDone Booleanpsl=}
```
We can then define a new `crud` called `Tasks`.
We specify to use the `Task` entity and we enable the `getAll`, `get`, `create` and `update` operations (let's say we don't need the `delete` operation).
main.wasp
```
crud Tasks { entity: Task, operations: { getAll: { isPublic: true, // by default only logged in users can perform operations }, get: {}, create: { overrideFn: import { createTask } from "@src/tasks.js", }, update: {}, },}
```
1. It uses default implementation for `getAll`, `get`, and `update`,
2. ... while specifying a custom implementation for `create`.
3. `getAll` will be public (no auth needed), while the rest of the operations will be private.
Here's what it looks like when visualized:
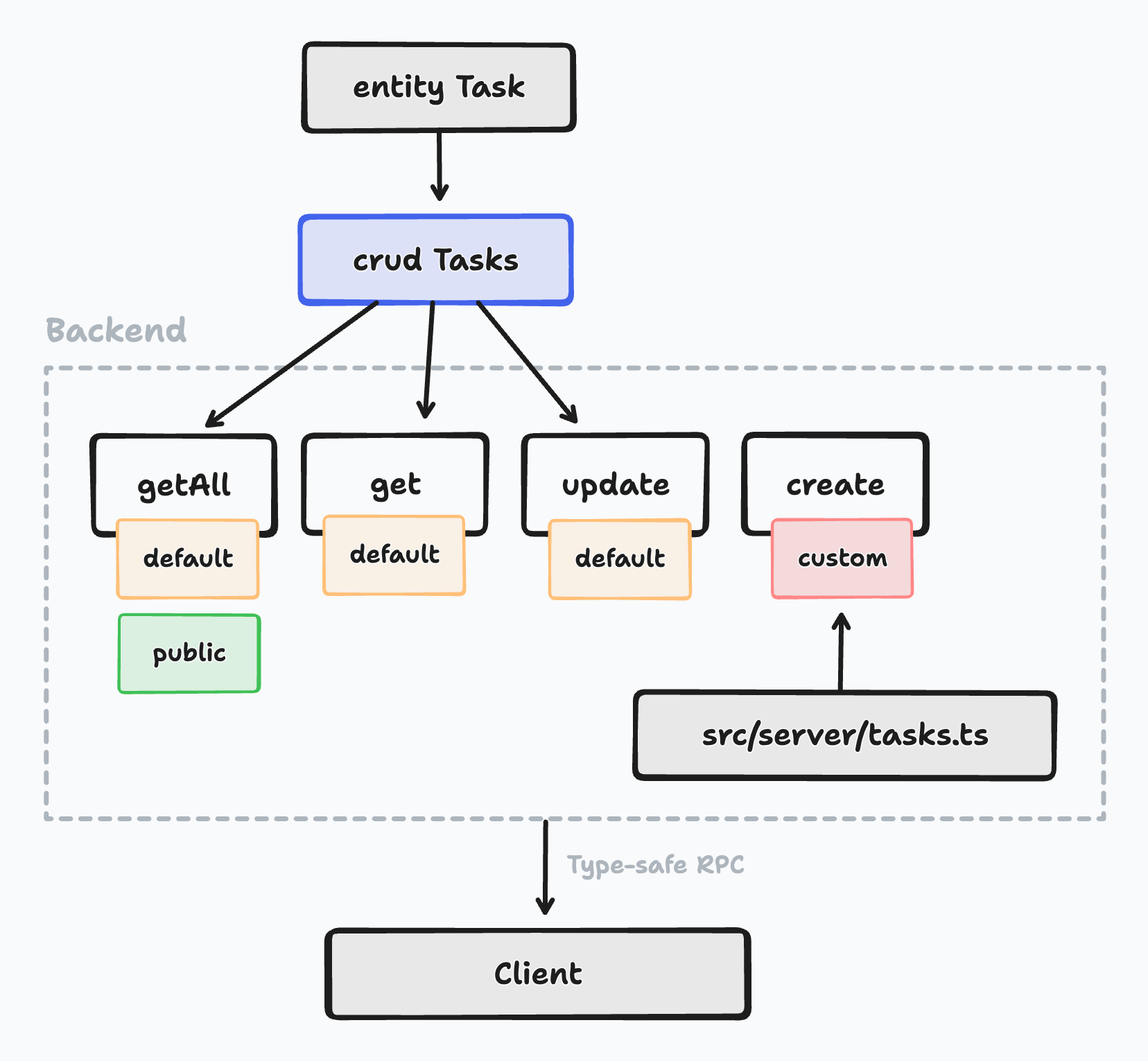
Visualization of the Tasks crud declaration
We can now use the CRUD queries and actions we just specified in our client code.
Keep reading for an example of Automatic CRUD in action, or skip ahead for the [API Reference](#api-reference)
## Example: A Simple TODO App[](#example-a-simple-todo-app "Direct link to Example: A Simple TODO App")
Let's create a full-app example that uses automatic CRUD. We'll stick to using the `Task` entity from the previous example, but we'll add a `User` entity and enable [username and password](https://wasp-lang.dev/docs/auth/username-and-pass) based auth.
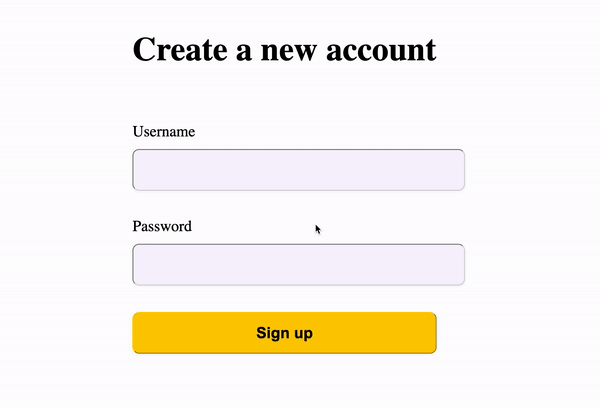
We are building a simple tasks app with username based auth
### Creating the App[](#creating-the-app "Direct link to Creating the App")
We can start by running `wasp new tasksCrudApp` and then adding the following to the `main.wasp` file:
main.wasp
```
app tasksCrudApp { wasp: { version: "^0.13.0" }, title: "Tasks Crud App", // We enabled auth and set the auth method to username and password auth: { userEntity: User, methods: { usernameAndPassword: {}, }, onAuthFailedRedirectTo: "/login", },}entity User {=psl id Int @id @default(autoincrement()) tasks Task[]psl=}// We defined a Task entity on which we'll enable CRUD later onentity Task {=psl id Int @id @default(autoincrement()) description String isDone Boolean userId Int user User @relation(fields: [userId], references: [id])psl=}// Tasks app routesroute RootRoute { path: "/", to: MainPage }page MainPage { component: import { MainPage } from "@src/MainPage.jsx", authRequired: true,}route LoginRoute { path: "/login", to: LoginPage }page LoginPage { component: import { LoginPage } from "@src/LoginPage.jsx",}route SignupRoute { path: "/signup", to: SignupPage }page SignupPage { component: import { SignupPage } from "@src/SignupPage.jsx",}
```
We can then run `wasp db migrate-dev` to create the database and run the migrations.
### Adding CRUD to the `Task` Entity ✨[](#adding-crud-to-the-task-entity- "Direct link to adding-crud-to-the-task-entity-")
Let's add the following `crud` declaration to our `main.wasp` file:
main.wasp
```
// ...crud Tasks { entity: Task, operations: { getAll: {}, create: { overrideFn: import { createTask } from "@src/tasks.js", }, },}
```
You'll notice that we enabled only `getAll` and `create` operations. This means that only these operations will be available.
We also overrode the `create` operation with a custom implementation. This means that the `create` operation will not be generated, but instead, the `createTask` function from `@src/tasks.js` will be used.
### Our Custom `create` Operation[](#our-custom-create-operation "Direct link to our-custom-create-operation")
Here's the `src/tasks.ts` file:
* JavaScript
* TypeScript
src/tasks.js
```
import { HttpError } from 'wasp/server'export const createTask = async (args, context) => { if (!context.user) { throw new HttpError(401, 'User not authenticated.') } const { description, isDone } = args const { Task } = context.entities return await Task.create({ data: { description, isDone, // Connect the task to the user that is creating it user: { connect: { id: context.user.id, }, }, }, })}
```
We made a custom `create` operation because we want to make sure that the task is connected to the user that is creating it. Automatic CRUD doesn't support this by default (yet!). Read more about the default implementations [here](#declaring-a-crud-with-default-options).
### Using the Generated CRUD Operations on the Client[](#using-the-generated-crud-operations-on-the-client "Direct link to Using the Generated CRUD Operations on the Client")
And let's use the generated operations in our client code:
* JavaScript
* TypeScript
src/MainPage.jsx
```
import { Tasks } from 'wasp/client/crud'import { useState } from 'react'export const MainPage = () => { const { data: tasks, isLoading, error } = Tasks.getAll.useQuery() const createTask = Tasks.create.useAction() const [taskDescription, setTaskDescription] = useState('') function handleCreateTask() { createTask({ description: taskDescription, isDone: false }) setTaskDescription('') } if (isLoading) return <div>Loading...</div> if (error) return <div>Error: {error.message}</div> return ( <div style={{ fontSize: '1.5rem', display: 'grid', placeContent: 'center', height: '100vh', }} > <div> <input value={taskDescription} onChange={(e) => setTaskDescription(e.target.value)} /> <button onClick={handleCreateTask}>Create task</button> </div> <ul> {tasks.map((task) => ( <li key={task.id}>{task.description}</li> ))} </ul> </div> )}
```
And here are the login and signup pages, where we are using Wasp's [Auth UI](https://wasp-lang.dev/docs/auth/ui) components:
* JavaScript
* TypeScript
src/LoginPage.jsx
```
import { LoginForm } from 'wasp/client/auth'import { Link } from 'react-router-dom'export function LoginPage() { return ( <div style={{ display: 'grid', placeContent: 'center', }} > <LoginForm /> <div> <Link to="/signup">Create an account</Link> </div> </div> )}
```
* JavaScript
* TypeScript
src/SignupPage.jsx
```
import { SignupForm } from 'wasp/client/auth'export function SignupPage() { return ( <div style={{ display: 'grid', placeContent: 'center', }} > <SignupForm /> </div> )}
```
That's it. You can now run `wasp start` and see the app in action. ⚡️
You should see a login page and a signup page. After you log in, you should see a page with a list of tasks and a form to create new tasks.
## Future of CRUD Operations in Wasp[](#future-of-crud-operations-in-wasp "Direct link to Future of CRUD Operations in Wasp")
CRUD operations currently have a limited set of knowledge about the business logic they are implementing.
* For example, they don't know that a task should be connected to the user that is creating it. This is why we had to override the `create` operation in the example above.
* Another thing: they are not aware of the authorization rules. For example, they don't know that a user should not be able to create a task for another user. In the future, we will be adding role-based authorization to Wasp, and we plan to make CRUD operations aware of the authorization rules.
* Another issue is input validation and sanitization. For example, we might want to make sure that the task description is not empty.
CRUD operations are a mechanism for getting a backend up and running quickly, but it depends on the information it can get from the Wasp app. The more information that it can pick up from your app, the more powerful it will be out of the box.
We plan on supporting CRUD operations and growing them to become the easiest way to create your backend. Follow along on [this GitHub issue](https://github.com/wasp-lang/wasp/issues/1253) to see how we are doing.
## API Reference[](#api-reference "Direct link to API Reference")
CRUD declaration work on top of existing entity declaration. We'll fully explore the API using two examples:
1. A basic CRUD declaration that relies on default options.
2. A more involved CRUD declaration that uses extra options and overrides.
### Declaring a CRUD With Default Options[](#declaring-a-crud-with-default-options "Direct link to Declaring a CRUD With Default Options")
If we create CRUD operations for an entity named `Task`, like this:
* JavaScript
* TypeScript
main.wasp
```
crud Tasks { // crud name here is "Tasks" entity: Task, operations: { get: {}, getAll: {}, create: {}, update: {}, delete: {}, },}
```
Wasp will give you the following default implementations:
**get** - returns one entity based on the `id` field
```
// ...// Wasp uses the field marked with `@id` in Prisma schema as the id field.return Task.findUnique({ where: { id: args.id } })
```
**getAll** - returns all entities
```
// ...// If the operation is not public, Wasp checks if an authenticated user// is making the request.return Task.findMany()
```
**create** - creates a new entity
```
// ...return Task.create({ data: args.data })
```
**update** - updates an existing entity
```
// ...// Wasp uses the field marked with `@id` in Prisma schema as the id field.return Task.update({ where: { id: args.id }, data: args.data })
```
**delete** - deletes an existing entity
```
// ...// Wasp uses the field marked with `@id` in Prisma schema as the id field.return Task.delete({ where: { id: args.id } })
```
Current Limitations
In the default `create` and `update` implementations, we are saving all of the data that the client sends to the server. This is not always desirable, i.e. in the case when the client should not be able to modify all of the data in the entity.
[In the future](#future-of-crud-operations-in-wasp), we are planning to add validation of action input, where only the data that the user is allowed to change will be saved.
For now, the solution is to provide an override function. You can override the default implementation by using the `overrideFn` option and implementing the validation logic yourself.
### Declaring a CRUD With All Available Options[](#declaring-a-crud-with-all-available-options "Direct link to Declaring a CRUD With All Available Options")
Here's an example of a more complex CRUD declaration:
* JavaScript
* TypeScript
main.wasp
```
crud Tasks { // crud name here is "Tasks" entity: Task, operations: { getAll: { isPublic: true, // optional, defaults to false }, get: {}, create: { overrideFn: import { createTask } from "@src/tasks.js", // optional }, update: {}, },}
```
The CRUD declaration features the following fields:
* `entity: Entity` required
The entity to which the CRUD operations will be applied.
* `operations: { [operationName]: CrudOperationOptions }` required
The operations to be generated. The key is the name of the operation, and the value is the operation configuration.
* The possible values for `operationName` are:
* `getAll`
* `get`
* `create`
* `update`
* `delete`
* `CrudOperationOptions` can have the following fields:
* `isPublic: bool` - Whether the operation is public or not. If it is public, no auth is required to access it. If it is not public, it will be available only to authenticated users. Defaults to `false`.
* `overrideFn: ExtImport` - The import statement of the optional override implementation in Node.js.
#### Defining the overrides[](#defining-the-overrides "Direct link to Defining the overrides")
Like with actions and queries, you can define the implementation in a Javascript/Typescript file. The overrides are functions that take the following arguments:
* `args`
The arguments of the operation i.e. the data sent from the client.
* `context`
Context contains the `user` making the request and the `entities` object with the entity that's being operated on.
For a usage example, check the [example guide](https://wasp-lang.dev/docs/data-model/crud#adding-crud-to-the-task-entity-).
#### Using the CRUD operations in client code[](#using-the-crud-operations-in-client-code "Direct link to Using the CRUD operations in client code")
On the client, you import the CRUD operations from `wasp/client/crud` by import the `{crud name}` object. For example, if you have a CRUD called `Tasks`, you would import the operations like this:
* JavaScript
* TypeScript
SomePage.jsx
```
import { Tasks } from 'wasp/client/crud'
```
You can then access the operations like this:
* JavaScript
* TypeScript
SomePage.jsx
```
const { data } = Tasks.getAll.useQuery()const { data } = Tasks.get.useQuery({ id: 1 })const createAction = Tasks.create.useAction()const updateAction = Tasks.update.useAction()const deleteAction = Tasks.delete.useAction()
```
All CRUD operations are implemented with [Queries and Actions](https://wasp-lang.dev/docs/data-model/operations/overview) under the hood, which means they come with all the features you'd expect (e.g., automatic SuperJSON serialization, full-stack type safety when using TypeScript)
* * *
Join our **community** on [Discord](https://discord.com/invite/rzdnErX), where we chat about full-stack web stuff. Join us to see what we are up to, share your opinions or get help with CRUD operations. | null | https://wasp-lang.dev/docs/data-model/crud | If you have a lot of experience writing full-stack apps, you probably ended up doing some of the same things many times: listing data, adding data, editing it, and deleting it. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecc721f7081cd-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:13:37 GMT | Tue, 23 Apr 2024 15:23:37 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=4H0i%2FirpQTSvzFXy2qIhJSw6zFByN1heoblqB6KN8WqyUPJk3lKb31e66ZYVYzDs9SDia5ZFqrCzlD5VzOcYk0oMlYJOiBaH9nbMpFvCgilAxFwXSbY1CXR6szVQUjc8"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | d3cbd1cc80ea60ba0fb87097f00892cd6d835c99 | null | 2380:52242:396FAF9:447557D:6627D021 | HIT | MISS | cache-iad-kiad7000118-IAD | S1713885218.634569,VS0,VE18 | null | null | en | og:image | https://wasp-lang.dev/docs/data-model/crud | og:url | Automatic CRUD | Wasp | og:title | If you have a lot of experience writing full-stack apps, you probably ended up doing some of the same things many times: listing data, adding data, editing it, and deleting it. | og:description | Automatic CRUD | Wasp | null | Automatic CRUD
If you have a lot of experience writing full-stack apps, you probably ended up doing some of the same things many times: listing data, adding data, editing it, and deleting it.
Wasp makes handling these boring bits easy by offering a higher-level concept called Automatic CRUD.
With a single declaration, you can tell Wasp to automatically generate server-side logic (i.e., Queries and Actions) for creating, reading, updating and deleting Entities. As you update definitions for your Entities, Wasp automatically regenerates the backend logic.
Early preview
This feature is currently in early preview and we are actively working on it. Read more about our plans for CRUD operations.
Overview
Imagine we have a Task entity and we want to enable CRUD operations for it.
main.wasp
entity Task {=psl
id Int @id @default(autoincrement())
description String
isDone Boolean
psl=}
We can then define a new crud called Tasks.
We specify to use the Task entity and we enable the getAll, get, create and update operations (let's say we don't need the delete operation).
main.wasp
crud Tasks {
entity: Task,
operations: {
getAll: {
isPublic: true, // by default only logged in users can perform operations
},
get: {},
create: {
overrideFn: import { createTask } from "@src/tasks.js",
},
update: {},
},
}
It uses default implementation for getAll, get, and update,
... while specifying a custom implementation for create.
getAll will be public (no auth needed), while the rest of the operations will be private.
Here's what it looks like when visualized:
Visualization of the Tasks crud declaration
We can now use the CRUD queries and actions we just specified in our client code.
Keep reading for an example of Automatic CRUD in action, or skip ahead for the API Reference
Example: A Simple TODO App
Let's create a full-app example that uses automatic CRUD. We'll stick to using the Task entity from the previous example, but we'll add a User entity and enable username and password based auth.
We are building a simple tasks app with username based auth
Creating the App
We can start by running wasp new tasksCrudApp and then adding the following to the main.wasp file:
main.wasp
app tasksCrudApp {
wasp: {
version: "^0.13.0"
},
title: "Tasks Crud App",
// We enabled auth and set the auth method to username and password
auth: {
userEntity: User,
methods: {
usernameAndPassword: {},
},
onAuthFailedRedirectTo: "/login",
},
}
entity User {=psl
id Int @id @default(autoincrement())
tasks Task[]
psl=}
// We defined a Task entity on which we'll enable CRUD later on
entity Task {=psl
id Int @id @default(autoincrement())
description String
isDone Boolean
userId Int
user User @relation(fields: [userId], references: [id])
psl=}
// Tasks app routes
route RootRoute { path: "/", to: MainPage }
page MainPage {
component: import { MainPage } from "@src/MainPage.jsx",
authRequired: true,
}
route LoginRoute { path: "/login", to: LoginPage }
page LoginPage {
component: import { LoginPage } from "@src/LoginPage.jsx",
}
route SignupRoute { path: "/signup", to: SignupPage }
page SignupPage {
component: import { SignupPage } from "@src/SignupPage.jsx",
}
We can then run wasp db migrate-dev to create the database and run the migrations.
Adding CRUD to the Task Entity ✨
Let's add the following crud declaration to our main.wasp file:
main.wasp
// ...
crud Tasks {
entity: Task,
operations: {
getAll: {},
create: {
overrideFn: import { createTask } from "@src/tasks.js",
},
},
}
You'll notice that we enabled only getAll and create operations. This means that only these operations will be available.
We also overrode the create operation with a custom implementation. This means that the create operation will not be generated, but instead, the createTask function from @src/tasks.js will be used.
Our Custom create Operation
Here's the src/tasks.ts file:
JavaScript
TypeScript
src/tasks.js
import { HttpError } from 'wasp/server'
export const createTask = async (args, context) => {
if (!context.user) {
throw new HttpError(401, 'User not authenticated.')
}
const { description, isDone } = args
const { Task } = context.entities
return await Task.create({
data: {
description,
isDone,
// Connect the task to the user that is creating it
user: {
connect: {
id: context.user.id,
},
},
},
})
}
We made a custom create operation because we want to make sure that the task is connected to the user that is creating it. Automatic CRUD doesn't support this by default (yet!). Read more about the default implementations here.
Using the Generated CRUD Operations on the Client
And let's use the generated operations in our client code:
JavaScript
TypeScript
src/MainPage.jsx
import { Tasks } from 'wasp/client/crud'
import { useState } from 'react'
export const MainPage = () => {
const { data: tasks, isLoading, error } = Tasks.getAll.useQuery()
const createTask = Tasks.create.useAction()
const [taskDescription, setTaskDescription] = useState('')
function handleCreateTask() {
createTask({ description: taskDescription, isDone: false })
setTaskDescription('')
}
if (isLoading) return <div>Loading...</div>
if (error) return <div>Error: {error.message}</div>
return (
<div
style={{
fontSize: '1.5rem',
display: 'grid',
placeContent: 'center',
height: '100vh',
}}
>
<div>
<input
value={taskDescription}
onChange={(e) => setTaskDescription(e.target.value)}
/>
<button onClick={handleCreateTask}>Create task</button>
</div>
<ul>
{tasks.map((task) => (
<li key={task.id}>{task.description}</li>
))}
</ul>
</div>
)
}
And here are the login and signup pages, where we are using Wasp's Auth UI components:
JavaScript
TypeScript
src/LoginPage.jsx
import { LoginForm } from 'wasp/client/auth'
import { Link } from 'react-router-dom'
export function LoginPage() {
return (
<div
style={{
display: 'grid',
placeContent: 'center',
}}
>
<LoginForm />
<div>
<Link to="/signup">Create an account</Link>
</div>
</div>
)
}
JavaScript
TypeScript
src/SignupPage.jsx
import { SignupForm } from 'wasp/client/auth'
export function SignupPage() {
return (
<div
style={{
display: 'grid',
placeContent: 'center',
}}
>
<SignupForm />
</div>
)
}
That's it. You can now run wasp start and see the app in action. ⚡️
You should see a login page and a signup page. After you log in, you should see a page with a list of tasks and a form to create new tasks.
Future of CRUD Operations in Wasp
CRUD operations currently have a limited set of knowledge about the business logic they are implementing.
For example, they don't know that a task should be connected to the user that is creating it. This is why we had to override the create operation in the example above.
Another thing: they are not aware of the authorization rules. For example, they don't know that a user should not be able to create a task for another user. In the future, we will be adding role-based authorization to Wasp, and we plan to make CRUD operations aware of the authorization rules.
Another issue is input validation and sanitization. For example, we might want to make sure that the task description is not empty.
CRUD operations are a mechanism for getting a backend up and running quickly, but it depends on the information it can get from the Wasp app. The more information that it can pick up from your app, the more powerful it will be out of the box.
We plan on supporting CRUD operations and growing them to become the easiest way to create your backend. Follow along on this GitHub issue to see how we are doing.
API Reference
CRUD declaration work on top of existing entity declaration. We'll fully explore the API using two examples:
A basic CRUD declaration that relies on default options.
A more involved CRUD declaration that uses extra options and overrides.
Declaring a CRUD With Default Options
If we create CRUD operations for an entity named Task, like this:
JavaScript
TypeScript
main.wasp
crud Tasks { // crud name here is "Tasks"
entity: Task,
operations: {
get: {},
getAll: {},
create: {},
update: {},
delete: {},
},
}
Wasp will give you the following default implementations:
get - returns one entity based on the id field
// ...
// Wasp uses the field marked with `@id` in Prisma schema as the id field.
return Task.findUnique({ where: { id: args.id } })
getAll - returns all entities
// ...
// If the operation is not public, Wasp checks if an authenticated user
// is making the request.
return Task.findMany()
create - creates a new entity
// ...
return Task.create({ data: args.data })
update - updates an existing entity
// ...
// Wasp uses the field marked with `@id` in Prisma schema as the id field.
return Task.update({ where: { id: args.id }, data: args.data })
delete - deletes an existing entity
// ...
// Wasp uses the field marked with `@id` in Prisma schema as the id field.
return Task.delete({ where: { id: args.id } })
Current Limitations
In the default create and update implementations, we are saving all of the data that the client sends to the server. This is not always desirable, i.e. in the case when the client should not be able to modify all of the data in the entity.
In the future, we are planning to add validation of action input, where only the data that the user is allowed to change will be saved.
For now, the solution is to provide an override function. You can override the default implementation by using the overrideFn option and implementing the validation logic yourself.
Declaring a CRUD With All Available Options
Here's an example of a more complex CRUD declaration:
JavaScript
TypeScript
main.wasp
crud Tasks { // crud name here is "Tasks"
entity: Task,
operations: {
getAll: {
isPublic: true, // optional, defaults to false
},
get: {},
create: {
overrideFn: import { createTask } from "@src/tasks.js", // optional
},
update: {},
},
}
The CRUD declaration features the following fields:
entity: Entity required
The entity to which the CRUD operations will be applied.
operations: { [operationName]: CrudOperationOptions } required
The operations to be generated. The key is the name of the operation, and the value is the operation configuration.
The possible values for operationName are:
getAll
get
create
update
delete
CrudOperationOptions can have the following fields:
isPublic: bool - Whether the operation is public or not. If it is public, no auth is required to access it. If it is not public, it will be available only to authenticated users. Defaults to false.
overrideFn: ExtImport - The import statement of the optional override implementation in Node.js.
Defining the overrides
Like with actions and queries, you can define the implementation in a Javascript/Typescript file. The overrides are functions that take the following arguments:
args
The arguments of the operation i.e. the data sent from the client.
context
Context contains the user making the request and the entities object with the entity that's being operated on.
For a usage example, check the example guide.
Using the CRUD operations in client code
On the client, you import the CRUD operations from wasp/client/crud by import the {crud name} object. For example, if you have a CRUD called Tasks, you would import the operations like this:
JavaScript
TypeScript
SomePage.jsx
import { Tasks } from 'wasp/client/crud'
You can then access the operations like this:
JavaScript
TypeScript
SomePage.jsx
const { data } = Tasks.getAll.useQuery()
const { data } = Tasks.get.useQuery({ id: 1 })
const createAction = Tasks.create.useAction()
const updateAction = Tasks.update.useAction()
const deleteAction = Tasks.delete.useAction()
All CRUD operations are implemented with Queries and Actions under the hood, which means they come with all the features you'd expect (e.g., automatic SuperJSON serialization, full-stack type safety when using TypeScript)
Join our community on Discord, where we chat about full-stack web stuff. Join us to see what we are up to, share your opinions or get help with CRUD operations. | https://wasp-lang.dev/docs/data-model/crud |
|
1 | null | 2024-04-23T15:13:40.823Z | https://wasp-lang.dev/docs/data-model/backends | https://wasp-lang.dev/docs | http | ## Databases
[Entities](https://wasp-lang.dev/docs/data-model/entities), [Operations](https://wasp-lang.dev/docs/data-model/operations/overview) and [Automatic CRUD](https://wasp-lang.dev/docs/data-model/crud) together make a high-level interface for working with your app's data. Still, all that data has to live somewhere, so let's see how Wasp deals with databases.
## Supported Database Backends[](#supported-database-backends "Direct link to Supported Database Backends")
Wasp supports multiple database backends. We'll list and explain each one.
### SQLite[](#sqlite "Direct link to SQLite")
The default database Wasp uses is [SQLite](https://www.sqlite.org/index.html).
SQLite is a great way for getting started with a new project because it doesn't require any configuration, but Wasp can only use it in development. Once you want to deploy your Wasp app to production, you'll need to switch to PostgreSQL and stick with it.
Fortunately, migrating from SQLite to PostgreSQL is pretty simple, and we have [a guide](#migrating-from-sqlite-to-postgresql) to help you.
### PostgreSQL[](#postgresql "Direct link to PostgreSQL")
[PostgreSQL](https://www.postgresql.org/) is the most advanced open-source database and one of the most popular databases overall. It's been in active development for 20+ years. Therefore, if you're looking for a battle-tested database, look no further.
To use Wasp with PostgreSQL, you'll have to ensure a database instance is running during development. Wasp needs access to your database for commands such as `wasp start` or `wasp db migrate-dev` and expects to find a connection string in the `DATABASE_URL` environment variable.
We cover all supported ways of connecting to a database in [the next section](#connecting-to-a-database).
### Migrating from SQLite to PostgreSQL[](#migrating-from-sqlite-to-postgresql "Direct link to Migrating from SQLite to PostgreSQL")
To run your Wasp app in production, you'll need to switch from SQLite to PostgreSQL.
1. Set the `app.db.system` field to PostgreSQL.
main.wasp
```
app MyApp { title: "My app", // ... db: { system: PostgreSQL, // ... }}
```
2. Delete all the old migrations, since they are SQLite migrations and can't be used with PostgreSQL, as well as the SQLite database by running [`wasp clean`](https://wasp-lang.dev/docs/general/cli#project-commands):
```
rm -r migrations/wasp clean
```
3. Ensure your new database is running (check the [section on connecting to a database](#connecting-to-a-database) to see how). Leave it running, since we need it for the next step.
4. In a different terminal, run `wasp db migrate-dev` to apply the changes and create a new initial migration.
5. That is it, you are all done!
## Connecting to a Database[](#connecting-to-a-database "Direct link to Connecting to a Database")
Assuming you're not using SQLite, Wasp offers two ways of connecting your app to a database instance:
1. A ready-made dev database that requires minimal setup and is great for quick prototyping.
2. A "real" database Wasp can connect to and use in production.
### Using the Dev Database Provided by Wasp[](#using-the-dev-database-provided-by-wasp "Direct link to Using the Dev Database Provided by Wasp")
The command `wasp start db` will start a default PostgreSQL dev database for you.
Your Wasp app will automatically connect to it, just keep `wasp start db` running in the background. Also, make sure that:
* You have [Docker installed](https://www.docker.com/get-started/) and in `PATH`.
* The port `5432` isn't taken.
### Connecting to an existing database[](#connecting-to-an-existing-database "Direct link to Connecting to an existing database")
If you want to spin up your own dev database (or connect to an external one), you can tell Wasp about it using the `DATABASE_URL` environment variable. Wasp will use the value of `DATABASE_URL` as a connection string.
The easiest way to set the necessary `DATABASE_URL` environment variable is by adding it to the [.env.server](https://wasp-lang.dev/docs/project/env-vars) file in the root dir of your Wasp project (if that file doesn't yet exist, create it).
Alternatively, you can set it inline when running `wasp` (this applies to all environment variables):
```
DATABASE_URL=<my-db-url> wasp ...
```
This trick is useful for running a certain `wasp` command on a specific database. For example, you could do:
```
DATABASE_URL=<production-db-url> wasp db seed myProductionSeed
```
This command seeds the data for a fresh staging or production database. To more precisely understand how seeding works, keep reading.
## Seeding the Database[](#seeding-the-database "Direct link to Seeding the Database")
**Database seeding** is a term used for populating the database with some initial data.
Seeding is most commonly used for two following scenarios:
1. To put the development database into a state convenient for working and testing.
2. To initialize any database (`dev`, `staging`, or `prod`) with essential data it requires to operate. For example, populating the Currency table with default currencies, or the Country table with all available countries.
### Writing a Seed Function[](#writing-a-seed-function "Direct link to Writing a Seed Function")
You can define as many **seed functions** as you want in an array under the `app.db.seeds` field:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { // ... db: { // ... seeds: [ import { devSeedSimple } from "@src/dbSeeds.js", import { prodSeed } from "@src/dbSeeds.js" ] }}
```
Each seed function must be an async function that takes one argument, `prisma`, which is a [Prisma Client](https://www.prisma.io/docs/concepts/components/prisma-client/crud) instance used to interact with the database. This is the same Prisma Client instance that Wasp uses internally and thus includes all of the usual features (e.g., password hashing).
Since a seed function falls under server-side code, it can import other server-side functions. This is convenient because you might want to seed the database using Actions.
Here's an example of a seed function that imports an Action:
* JavaScript
* TypeScript
```
import { createTask } from './actions.js'import { sanitizeAndSerializeProviderData } from 'wasp/server/auth'export const devSeedSimple = async (prisma) => { const user = await createUser(prisma, { username: 'RiuTheDog', password: 'bark1234', }) await createTask( { description: 'Chase the cat' }, { user, entities: { Task: prisma.task } } )}async function createUser(prisma, data) { const newUser = await prisma.user.create({ data: { auth: { create: { identities: { create: { providerName: 'username', providerUserId: data.username, providerData: sanitizeAndSerializeProviderData({ hashedPassword: data.password }), }, }, }, }, }, }) return newUser}
```
### Running seed functions[](#running-seed-functions "Direct link to Running seed functions")
Run the command `wasp db seed` and Wasp will ask you which seed function you'd like to run (if you've defined more than one).
Alternatively, run the command `wasp db seed <seed-name>` to choose a specific seed function right away, for example:
```
wasp db seed devSeedSimple
```
Check the [API Reference](#cli-commands-for-seeding-the-database) for more details on these commands.
tip
You'll often want to call `wasp db seed` right after you run `wasp db reset`, as it makes sense to fill the database with initial data after clearing it.
## Prisma Configuration[](#prisma-configuration "Direct link to Prisma Configuration")
Wasp uses [Prisma](https://www.prisma.io/) to interact with the database. Prisma is a "Next-generation Node.js and TypeScript ORM" that provides a type-safe API for working with your database.
### Prisma Preview Features[](#prisma-preview-features "Direct link to Prisma Preview Features")
Prisma is still in active development and some of its features are not yet stable. To use them, you have to enable them in the `app.db.prisma.clientPreviewFeatures` field:
main.wasp
```
app MyApp { // ... db: { system: PostgreSQL, prisma: { clientPreviewFeatures: ["postgresqlExtensions"] } }}
```
Read more about Prisma preview features in the [Prisma docs](https://www.prisma.io/docs/concepts/components/preview-features/client-preview-features).
### PostgreSQL Extensions[](#postgresql-extensions "Direct link to PostgreSQL Extensions")
PostgreSQL supports [extensions](https://www.postgresql.org/docs/current/contrib.html) that add additional functionality to the database. For example, the [hstore](https://www.postgresql.org/docs/13/hstore.html) extension adds support for storing key-value pairs in a single column.
To use PostgreSQL extensions with Prisma, you have to enable them in the `app.db.prisma.dbExtensions` field:
main.wasp
```
app MyApp { // ... db: { system: PostgreSQL, prisma: { clientPreviewFeatures: ["postgresqlExtensions"] dbExtensions: [ { name: "hstore", schema: "myHstoreSchema" }, { name: "pg_trgm" }, { name: "postgis", version: "2.1" }, ] } }}
```
Read more about PostgreSQL configuration in Wasp in the [API Reference](#the-appdb-field).
## API Reference[](#api-reference "Direct link to API Reference")
You can tell Wasp which database to use in the `app` declaration's `db` field:
### The `app.db` Field[](#the-appdb-field "Direct link to the-appdb-field")
Here's an example that uses the `app.db` field to its full potential:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { title: "My app", // ... db: { system: PostgreSQL, seeds: [ import devSeed from "@src/dbSeeds.js" ], prisma: { clientPreviewFeatures: ["extendedWhereUnique"] } }}
```
`app.db` is a dictionary with the following fields (all fields are optional):
* `system: DbSystem`
The database system Wasp should use. It can be either PostgreSQL or SQLite. The default value for the field is SQLite (this default value also applies if the entire `db` field is left unset). Whenever you modify the `db.system` field, make sure to run `wasp db migrate-dev` to apply the changes.
* `seeds: [ExtImport]`
Defines the seed functions you can use with the `wasp db seed` command to seed your database with initial data. Read the [Seeding section](#seeding-the-database) for more details.
* `prisma: PrismaOptions`
Additional configuration for Prisma.
main.wasp
```
app MyApp { // ... db: { // ... prisma: { clientPreviewFeatures: ["postgresqlExtensions"], dbExtensions: [ { name: "hstore", schema: "myHstoreSchema" }, { name: "pg_trgm" }, { name: "postgis", version: "2.1" }, ] } }}
```
It's a dictionary with the following fields:
* `clientPreviewFeatures : [string]`
Allows you to define [Prisma client preview features](https://www.prisma.io/docs/concepts/components/preview-features/client-preview-features), like for example, `"postgresqlExtensions"`.
* `dbExtensions: DbExtension[]`
It allows you to define PostgreSQL extensions that should be enabled for your database. Read more about [PostgreSQL extensions in Prisma](https://www.prisma.io/docs/concepts/components/prisma-schema/postgresql-extensions).
For each extension you define a dict with the following fields:
* `name: string` required
The name of the extension you would normally put in the Prisma file.
schema.prisma
```
extensions = [hstore(schema: "myHstoreSchema"), pg_trgm, postgis(version: "2.1")]// 👆 Extension name
```
* `map: string`
It sets the `map` argument of the extension. Explanation for the field from the Prisma docs:
> This is the database name of the extension. If this argument is not specified, the name of the extension in the Prisma schema must match the database name.
* `schema: string`
It sets the `schema` argument of the extension. Explanation for the field from the Prisma docs:
> This is the name of the schema in which to activate the extension's objects. If this argument is not specified, the current default object creation schema is used.
* `version: string`
It sets the `version` argument of the extension. Explanation for the field from the Prisma docs:
> This is the version of the extension to activate. If this argument is not specified, the value given in the extension's control file is used.
### CLI Commands for Seeding the Database[](#cli-commands-for-seeding-the-database "Direct link to CLI Commands for Seeding the Database")
Use one of the following commands to run the seed functions:
* `wasp db seed`
If you've only defined a single seed function, this command runs it. If you've defined multiple seed functions, it asks you to choose one interactively.
* `wasp db seed <seed-name>`
This command runs the seed function with the specified name. The name is the identifier used in its `import` expression in the `app.db.seeds` list. For example, to run the seed function `devSeedSimple` which was defined like this:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { // ... db: { // ... seeds: [ // ... import { devSeedSimple } from "@src/dbSeeds.js", ] }}
```
Use the following command:
```
wasp db seed devSeedSimple
``` | null | https://wasp-lang.dev/docs/data-model/backends | Entities, Operations and Automatic CRUD together make a high-level interface for working with your app's data. Still, all that data has to live somewhere, so let's see how Wasp deals with databases. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecc842c9e81b7-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:13:40 GMT | Tue, 23 Apr 2024 15:23:40 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=F3D9QAvttKQmHgSULoMD%2Fbepm9JUMrhCz2lGy6adw%2Bf%2FxTE1L%2FGYhXDIPWhrE8%2BOm%2FPbcRFGs9XSItyUB6WRVJf1AN%2Bo1FF1WliQ6B3AJ%2F3UOWKQK2dKHVJYadAh9odM"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | e8e3f25bebe7750f697e8818746a3fb7e852ec2a | null | F104:3F9EB3:3A37D48:453CB4D:6627D024 | HIT | MISS | cache-iad-kiad7000138-IAD | S1713885221.525737,VS0,VE14 | null | null | en | og:image | https://wasp-lang.dev/docs/data-model/backends | og:url | Databases | Wasp | og:title | Entities, Operations and Automatic CRUD together make a high-level interface for working with your app's data. Still, all that data has to live somewhere, so let's see how Wasp deals with databases. | og:description | Databases | Wasp | null | Databases
Entities, Operations and Automatic CRUD together make a high-level interface for working with your app's data. Still, all that data has to live somewhere, so let's see how Wasp deals with databases.
Supported Database Backends
Wasp supports multiple database backends. We'll list and explain each one.
SQLite
The default database Wasp uses is SQLite.
SQLite is a great way for getting started with a new project because it doesn't require any configuration, but Wasp can only use it in development. Once you want to deploy your Wasp app to production, you'll need to switch to PostgreSQL and stick with it.
Fortunately, migrating from SQLite to PostgreSQL is pretty simple, and we have a guide to help you.
PostgreSQL
PostgreSQL is the most advanced open-source database and one of the most popular databases overall. It's been in active development for 20+ years. Therefore, if you're looking for a battle-tested database, look no further.
To use Wasp with PostgreSQL, you'll have to ensure a database instance is running during development. Wasp needs access to your database for commands such as wasp start or wasp db migrate-dev and expects to find a connection string in the DATABASE_URL environment variable.
We cover all supported ways of connecting to a database in the next section.
Migrating from SQLite to PostgreSQL
To run your Wasp app in production, you'll need to switch from SQLite to PostgreSQL.
Set the app.db.system field to PostgreSQL.
main.wasp
app MyApp {
title: "My app",
// ...
db: {
system: PostgreSQL,
// ...
}
}
Delete all the old migrations, since they are SQLite migrations and can't be used with PostgreSQL, as well as the SQLite database by running wasp clean:
rm -r migrations/
wasp clean
Ensure your new database is running (check the section on connecting to a database to see how). Leave it running, since we need it for the next step.
In a different terminal, run wasp db migrate-dev to apply the changes and create a new initial migration.
That is it, you are all done!
Connecting to a Database
Assuming you're not using SQLite, Wasp offers two ways of connecting your app to a database instance:
A ready-made dev database that requires minimal setup and is great for quick prototyping.
A "real" database Wasp can connect to and use in production.
Using the Dev Database Provided by Wasp
The command wasp start db will start a default PostgreSQL dev database for you.
Your Wasp app will automatically connect to it, just keep wasp start db running in the background. Also, make sure that:
You have Docker installed and in PATH.
The port 5432 isn't taken.
Connecting to an existing database
If you want to spin up your own dev database (or connect to an external one), you can tell Wasp about it using the DATABASE_URL environment variable. Wasp will use the value of DATABASE_URL as a connection string.
The easiest way to set the necessary DATABASE_URL environment variable is by adding it to the .env.server file in the root dir of your Wasp project (if that file doesn't yet exist, create it).
Alternatively, you can set it inline when running wasp (this applies to all environment variables):
DATABASE_URL=<my-db-url> wasp ...
This trick is useful for running a certain wasp command on a specific database. For example, you could do:
DATABASE_URL=<production-db-url> wasp db seed myProductionSeed
This command seeds the data for a fresh staging or production database. To more precisely understand how seeding works, keep reading.
Seeding the Database
Database seeding is a term used for populating the database with some initial data.
Seeding is most commonly used for two following scenarios:
To put the development database into a state convenient for working and testing.
To initialize any database (dev, staging, or prod) with essential data it requires to operate. For example, populating the Currency table with default currencies, or the Country table with all available countries.
Writing a Seed Function
You can define as many seed functions as you want in an array under the app.db.seeds field:
JavaScript
TypeScript
main.wasp
app MyApp {
// ...
db: {
// ...
seeds: [
import { devSeedSimple } from "@src/dbSeeds.js",
import { prodSeed } from "@src/dbSeeds.js"
]
}
}
Each seed function must be an async function that takes one argument, prisma, which is a Prisma Client instance used to interact with the database. This is the same Prisma Client instance that Wasp uses internally and thus includes all of the usual features (e.g., password hashing).
Since a seed function falls under server-side code, it can import other server-side functions. This is convenient because you might want to seed the database using Actions.
Here's an example of a seed function that imports an Action:
JavaScript
TypeScript
import { createTask } from './actions.js'
import { sanitizeAndSerializeProviderData } from 'wasp/server/auth'
export const devSeedSimple = async (prisma) => {
const user = await createUser(prisma, {
username: 'RiuTheDog',
password: 'bark1234',
})
await createTask(
{ description: 'Chase the cat' },
{ user, entities: { Task: prisma.task } }
)
}
async function createUser(prisma, data) {
const newUser = await prisma.user.create({
data: {
auth: {
create: {
identities: {
create: {
providerName: 'username',
providerUserId: data.username,
providerData: sanitizeAndSerializeProviderData({
hashedPassword: data.password
}),
},
},
},
},
},
})
return newUser
}
Running seed functions
Run the command wasp db seed and Wasp will ask you which seed function you'd like to run (if you've defined more than one).
Alternatively, run the command wasp db seed <seed-name> to choose a specific seed function right away, for example:
wasp db seed devSeedSimple
Check the API Reference for more details on these commands.
tip
You'll often want to call wasp db seed right after you run wasp db reset, as it makes sense to fill the database with initial data after clearing it.
Prisma Configuration
Wasp uses Prisma to interact with the database. Prisma is a "Next-generation Node.js and TypeScript ORM" that provides a type-safe API for working with your database.
Prisma Preview Features
Prisma is still in active development and some of its features are not yet stable. To use them, you have to enable them in the app.db.prisma.clientPreviewFeatures field:
main.wasp
app MyApp {
// ...
db: {
system: PostgreSQL,
prisma: {
clientPreviewFeatures: ["postgresqlExtensions"]
}
}
}
Read more about Prisma preview features in the Prisma docs.
PostgreSQL Extensions
PostgreSQL supports extensions that add additional functionality to the database. For example, the hstore extension adds support for storing key-value pairs in a single column.
To use PostgreSQL extensions with Prisma, you have to enable them in the app.db.prisma.dbExtensions field:
main.wasp
app MyApp {
// ...
db: {
system: PostgreSQL,
prisma: {
clientPreviewFeatures: ["postgresqlExtensions"]
dbExtensions: [
{ name: "hstore", schema: "myHstoreSchema" },
{ name: "pg_trgm" },
{ name: "postgis", version: "2.1" },
]
}
}
}
Read more about PostgreSQL configuration in Wasp in the API Reference.
API Reference
You can tell Wasp which database to use in the app declaration's db field:
The app.db Field
Here's an example that uses the app.db field to its full potential:
JavaScript
TypeScript
main.wasp
app MyApp {
title: "My app",
// ...
db: {
system: PostgreSQL,
seeds: [
import devSeed from "@src/dbSeeds.js"
],
prisma: {
clientPreviewFeatures: ["extendedWhereUnique"]
}
}
}
app.db is a dictionary with the following fields (all fields are optional):
system: DbSystem
The database system Wasp should use. It can be either PostgreSQL or SQLite. The default value for the field is SQLite (this default value also applies if the entire db field is left unset). Whenever you modify the db.system field, make sure to run wasp db migrate-dev to apply the changes.
seeds: [ExtImport]
Defines the seed functions you can use with the wasp db seed command to seed your database with initial data. Read the Seeding section for more details.
prisma: PrismaOptions
Additional configuration for Prisma.
main.wasp
app MyApp {
// ...
db: {
// ...
prisma: {
clientPreviewFeatures: ["postgresqlExtensions"],
dbExtensions: [
{ name: "hstore", schema: "myHstoreSchema" },
{ name: "pg_trgm" },
{ name: "postgis", version: "2.1" },
]
}
}
}
It's a dictionary with the following fields:
clientPreviewFeatures : [string]
Allows you to define Prisma client preview features, like for example, "postgresqlExtensions".
dbExtensions: DbExtension[]
It allows you to define PostgreSQL extensions that should be enabled for your database. Read more about PostgreSQL extensions in Prisma.
For each extension you define a dict with the following fields:
name: string required
The name of the extension you would normally put in the Prisma file.
schema.prisma
extensions = [hstore(schema: "myHstoreSchema"), pg_trgm, postgis(version: "2.1")]
// 👆 Extension name
map: string
It sets the map argument of the extension. Explanation for the field from the Prisma docs:
This is the database name of the extension. If this argument is not specified, the name of the extension in the Prisma schema must match the database name.
schema: string
It sets the schema argument of the extension. Explanation for the field from the Prisma docs:
This is the name of the schema in which to activate the extension's objects. If this argument is not specified, the current default object creation schema is used.
version: string
It sets the version argument of the extension. Explanation for the field from the Prisma docs:
This is the version of the extension to activate. If this argument is not specified, the value given in the extension's control file is used.
CLI Commands for Seeding the Database
Use one of the following commands to run the seed functions:
wasp db seed
If you've only defined a single seed function, this command runs it. If you've defined multiple seed functions, it asks you to choose one interactively.
wasp db seed <seed-name>
This command runs the seed function with the specified name. The name is the identifier used in its import expression in the app.db.seeds list. For example, to run the seed function devSeedSimple which was defined like this:
JavaScript
TypeScript
main.wasp
app MyApp {
// ...
db: {
// ...
seeds: [
// ...
import { devSeedSimple } from "@src/dbSeeds.js",
]
}
}
Use the following command:
wasp db seed devSeedSimple | https://wasp-lang.dev/docs/data-model/backends |
|
1 | null | 2024-04-23T15:13:49.490Z | https://wasp-lang.dev/docs/auth/ui | https://wasp-lang.dev/docs | http | ## Auth UI
To make using authentication in your app as easy as possible, Wasp generates the server-side code but also the client-side UI for you. It enables you to quickly get the login, signup, password reset and email verification flows in your app.
Below we cover all of the available UI components and how to use them.
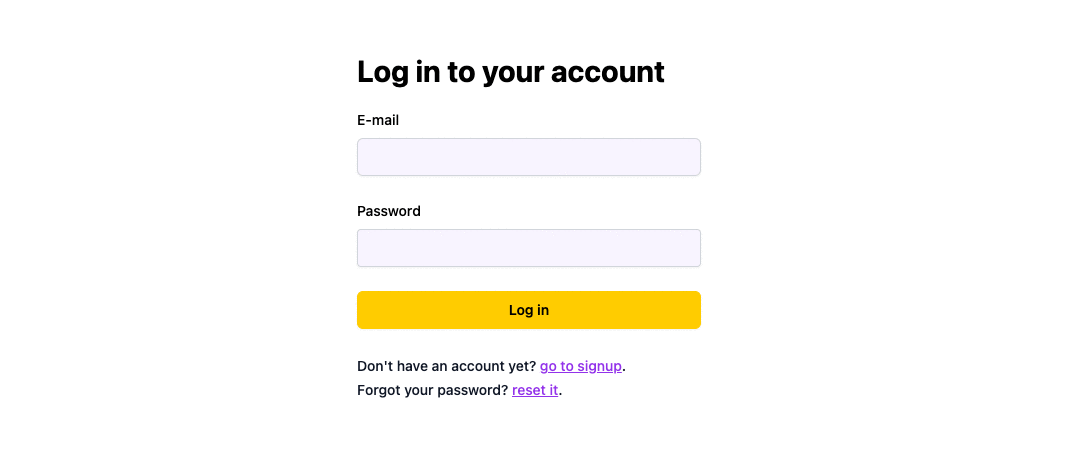
## Overview[](#overview "Direct link to Overview")
After Wasp generates the UI components for your auth, you can use it as is, or customize it to your liking.
Based on the authentication providers you enabled in your `main.wasp` file, the Auth UI will show the corresponding UI (form and buttons). For example, if you enabled e-mail authentication:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { //... auth: { methods: { email: {}, }, // ... }}
```
You'll get the following UI:
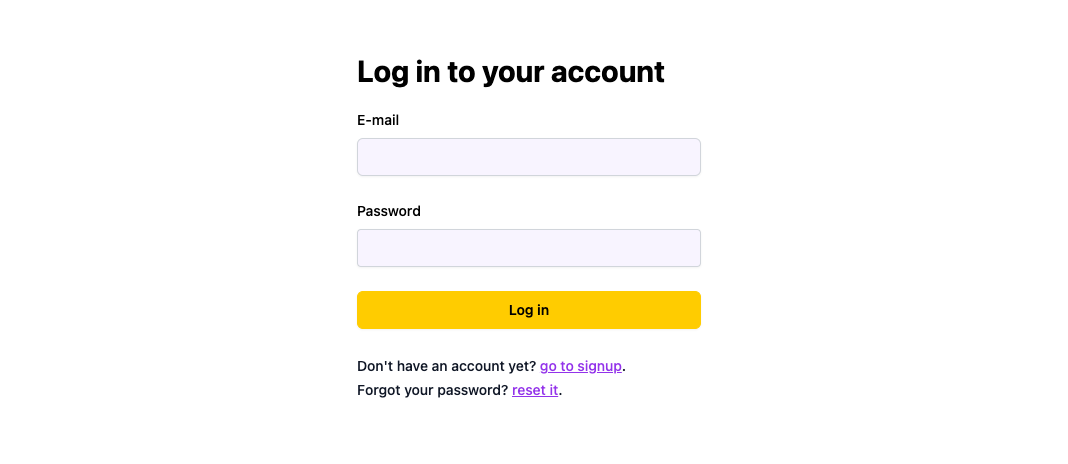
And then if you enable Google and Github:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { //... auth: { methods: { email: {}, google: {}, github: {}, }, // ... }}
```
The form will automatically update to look like this:
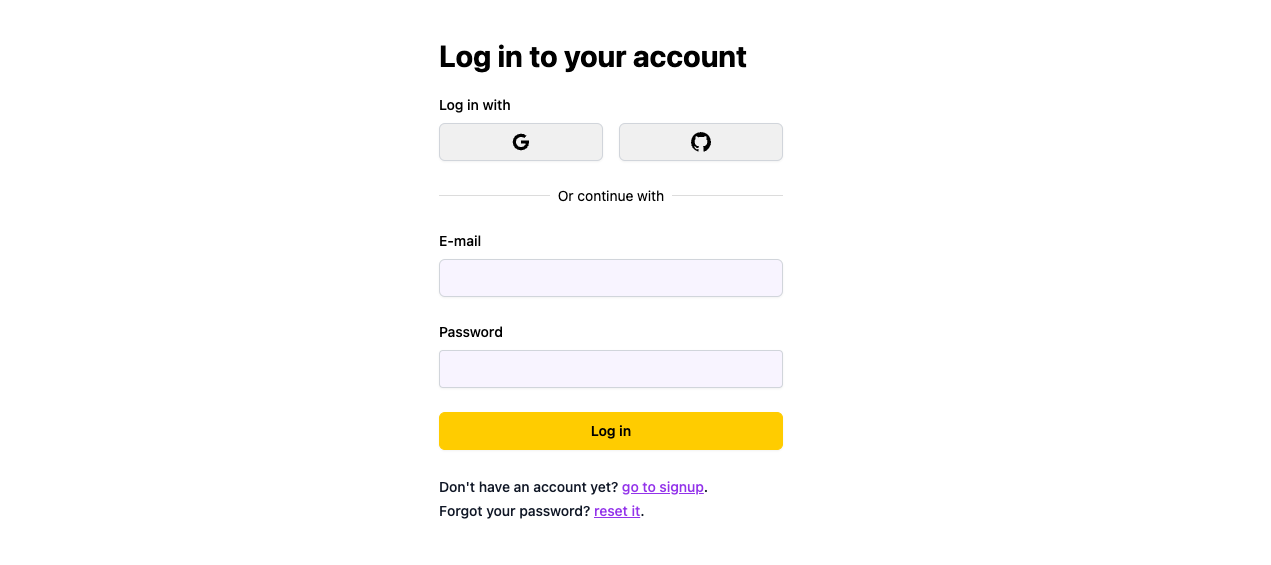
Let's go through all of the available components and how to use them.
## Auth Components[](#auth-components "Direct link to Auth Components")
The following components are available for you to use in your app:
* [Login form](#login-form)
* [Signup form](#signup-form)
* [Forgot password form](#forgot-password-form)
* [Reset password form](#reset-password-form)
* [Verify email form](#verify-email-form)
### Login Form[](#login-form "Direct link to Login Form")
Used with [Username & Password](https://wasp-lang.dev/docs/auth/username-and-pass), [Email](https://wasp-lang.dev/docs/auth/email), [Github](https://wasp-lang.dev/docs/auth/social-auth/github), [Google](https://wasp-lang.dev/docs/auth/social-auth/google) and [Keycloak](https://wasp-lang.dev/docs/auth/social-auth/keycloak) authentication.
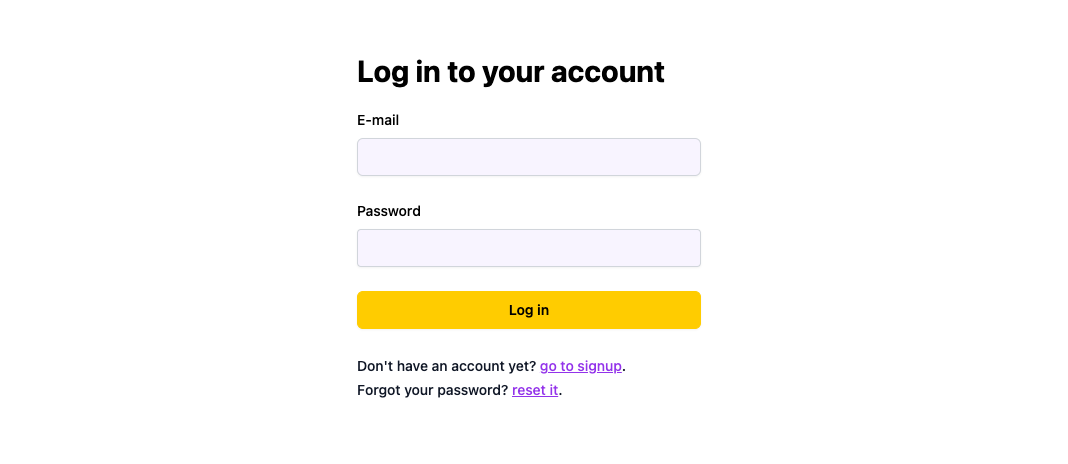
You can use the `LoginForm` component to build your login page:
* JavaScript
* TypeScript
main.wasp
```
// ...route LoginRoute { path: "/login", to: LoginPage }page LoginPage { component: import { LoginPage } from "@src/LoginPage.jsx"}
```
src/LoginPage.jsx
```
import { LoginForm } from 'wasp/client/auth'// Use it like thisexport function LoginPage() { return <LoginForm />}
```
It will automatically show the correct authentication providers based on your `main.wasp` file.
### Signup Form[](#signup-form "Direct link to Signup Form")
Used with [Username & Password](https://wasp-lang.dev/docs/auth/username-and-pass), [Email](https://wasp-lang.dev/docs/auth/email), [Github](https://wasp-lang.dev/docs/auth/social-auth/github), [Google](https://wasp-lang.dev/docs/auth/social-auth/google) and [Keycloak](https://wasp-lang.dev/docs/auth/social-auth/keycloak) authentication.
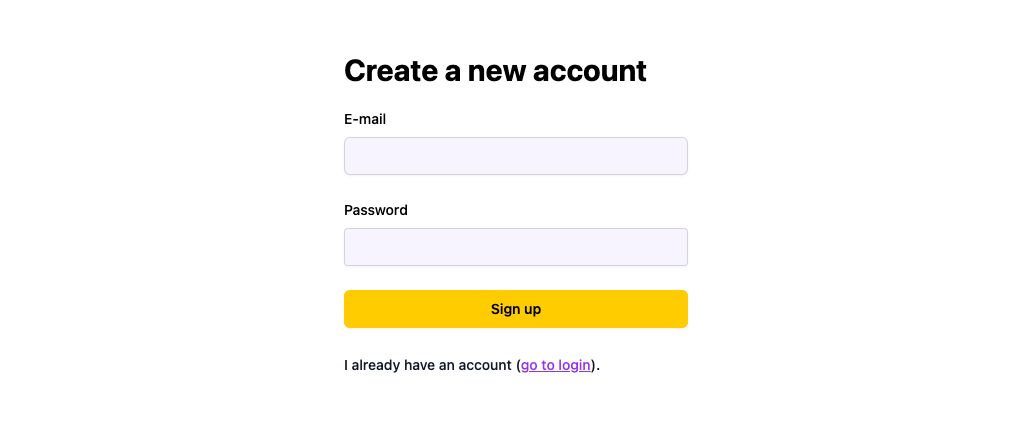
You can use the `SignupForm` component to build your signup page:
* JavaScript
* TypeScript
main.wasp
```
// ...route SignupRoute { path: "/signup", to: SignupPage }page SignupPage { component: import { SignupPage } from "@src/SignupPage.jsx"}
```
src/SignupPage.jsx
```
import { SignupForm } from 'wasp/client/auth'// Use it like thisexport function SignupPage() { return <SignupForm />}
```
It will automatically show the correct authentication providers based on your `main.wasp` file.
Read more about customizing the signup process like adding additional fields or extra UI in the [Auth Overview](https://wasp-lang.dev/docs/auth/overview#customizing-the-signup-process) section.
### Forgot Password Form[](#forgot-password-form "Direct link to Forgot Password Form")
Used with [Email](https://wasp-lang.dev/docs/auth/email) authentication.
If users forget their password, they can use this form to reset it.
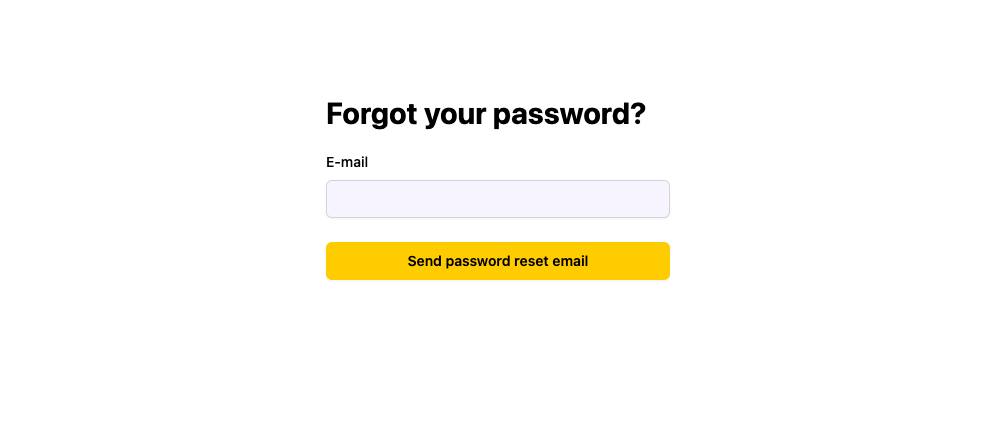
You can use the `ForgotPasswordForm` component to build your own forgot password page:
* JavaScript
* TypeScript
main.wasp
```
// ...route RequestPasswordResetRoute { path: "/request-password-reset", to: RequestPasswordResetPage }page RequestPasswordResetPage { component: import { ForgotPasswordPage } from "@src/ForgotPasswordPage.jsx"}
```
src/ForgotPasswordPage.jsx
```
import { ForgotPasswordForm } from 'wasp/client/auth'// Use it like thisexport function ForgotPasswordPage() { return <ForgotPasswordForm />}
```
### Reset Password Form[](#reset-password-form "Direct link to Reset Password Form")
Used with [Email](https://wasp-lang.dev/docs/auth/email) authentication.
After users click on the link in the email they receive after submitting the forgot password form, they will be redirected to this form where they can reset their password.
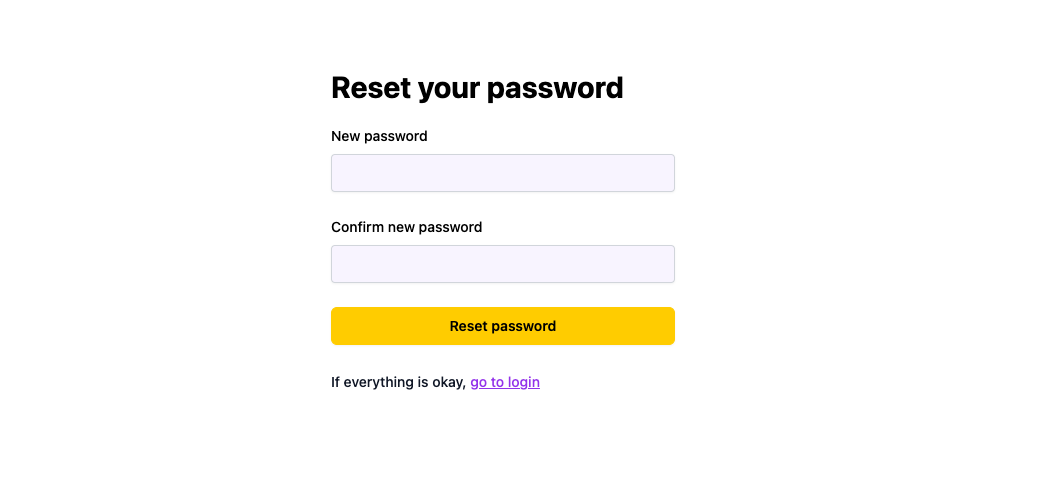
You can use the `ResetPasswordForm` component to build your reset password page:
* JavaScript
* TypeScript
main.wasp
```
// ...route PasswordResetRoute { path: "/password-reset", to: PasswordResetPage }page PasswordResetPage { component: import { ResetPasswordPage } from "@src/ResetPasswordPage.jsx"}
```
src/ResetPasswordPage.jsx
```
import { ResetPasswordForm } from 'wasp/client/auth'// Use it like thisexport function ResetPasswordPage() { return <ResetPasswordForm />}
```
### Verify Email Form[](#verify-email-form "Direct link to Verify Email Form")
Used with [Email](https://wasp-lang.dev/docs/auth/email) authentication.
After users sign up, they will receive an email with a link to this form where they can verify their email.
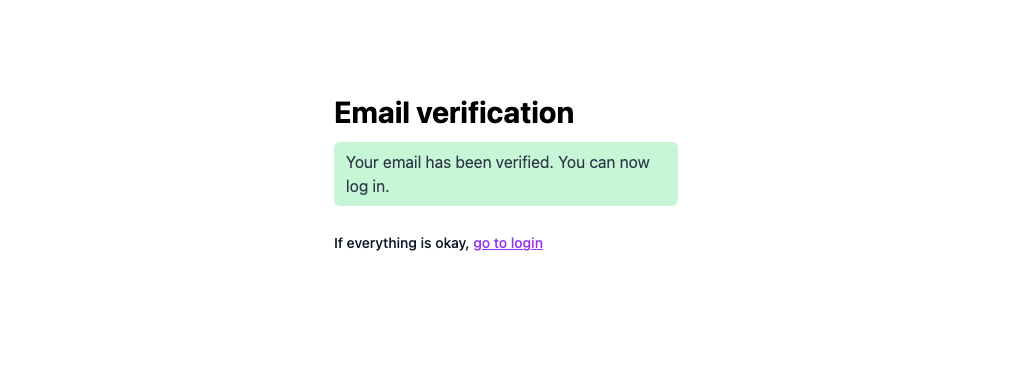
You can use the `VerifyEmailForm` component to build your email verification page:
* JavaScript
* TypeScript
main.wasp
```
// ...route EmailVerificationRoute { path: "/email-verification", to: EmailVerificationPage }page EmailVerificationPage { component: import { VerifyEmailPage } from "@src/VerifyEmailPage.jsx"}
```
src/VerifyEmailPage.jsx
```
import { VerifyEmailForm } from 'wasp/client/auth'// Use it like thisexport function VerifyEmailPage() { return <VerifyEmailForm />}
```
## Customization 💅🏻[](#customization- "Direct link to Customization 💅🏻")
You customize all of the available forms by passing props to them.
Props you can pass to all of the forms:
1. `appearance` - customize the form colors (via design tokens)
2. `logo` - path to your logo
3. `socialLayout` - layout of the social buttons, which can be `vertical` or `horizontal`
### 1\. Customizing the Colors[](#1-customizing-the-colors "Direct link to 1. Customizing the Colors")
We use [Stitches](https://stitches.dev/) to style the Auth UI. You can customize the styles by overriding the default theme tokens.
* JavaScript
* TypeScript
src/appearance.js
```
export const authAppearance = { colors: { brand: '#5969b8', // blue brandAccent: '#de5998', // pink submitButtonText: 'white', },}
```
src/LoginPage.jsx
```
import { LoginForm } from 'wasp/client/auth'import { authAppearance } from './appearance'export function LoginPage() { return ( <LoginForm // Pass the appearance object to the form appearance={authAppearance} /> )}
```
We recommend defining your appearance in a separate file and importing it into your components.
### 2\. Using Your Logo[](#2-using-your-logo "Direct link to 2. Using Your Logo")
You can add your logo to the Auth UI by passing the `logo` prop to any of the components.
* JavaScript
* TypeScript
src/LoginPage.jsx
```
import { LoginForm } from 'wasp/client/auth'import Logo from './logo.png'export function LoginPage() { return ( <LoginForm // Pass in the path to your logo logo={Logo} /> )}
```
You can change the layout of the social buttons by passing the `socialLayout` prop to any of the components. It can be either `vertical` or `horizontal` (default).
If we pass in `vertical`:
* JavaScript
* TypeScript
src/LoginPage.jsx
```
import { LoginForm } from 'wasp/client/auth'export function LoginPage() { return ( <LoginForm // Pass in the socialLayout prop socialLayout="vertical" /> )}
```
We get this:
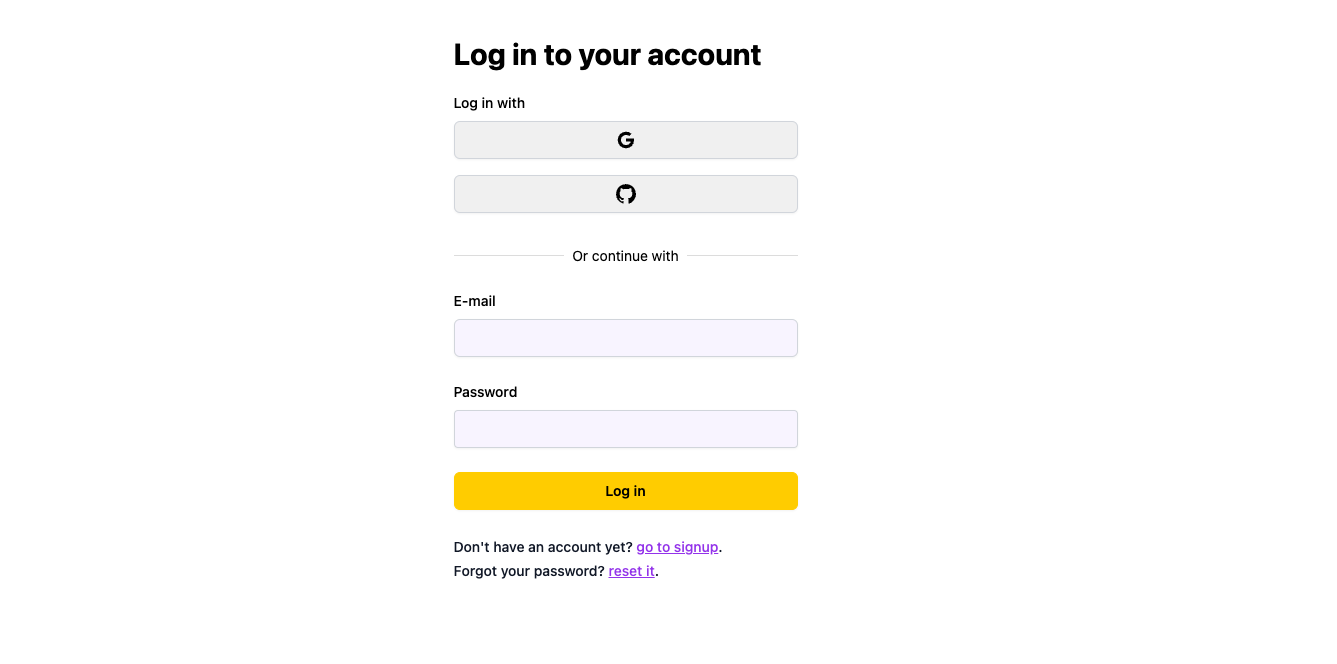
### Let's Put Everything Together 🪄[](#lets-put-everything-together- "Direct link to Let's Put Everything Together 🪄")
If we provide the logo and custom colors:
* JavaScript
* TypeScript
src/appearance.js
```
export const appearance = { colors: { brand: '#5969b8', // blue brandAccent: '#de5998', // pink submitButtonText: 'white', },}
```
src/LoginPage.jsx
```
import { LoginForm } from 'wasp/client/auth'import { authAppearance } from './appearance'import todoLogo from './todoLogo.png'export function LoginPage() { return <LoginForm appearance={appearance} logo={todoLogo} />}
```
We get a form looking like this:
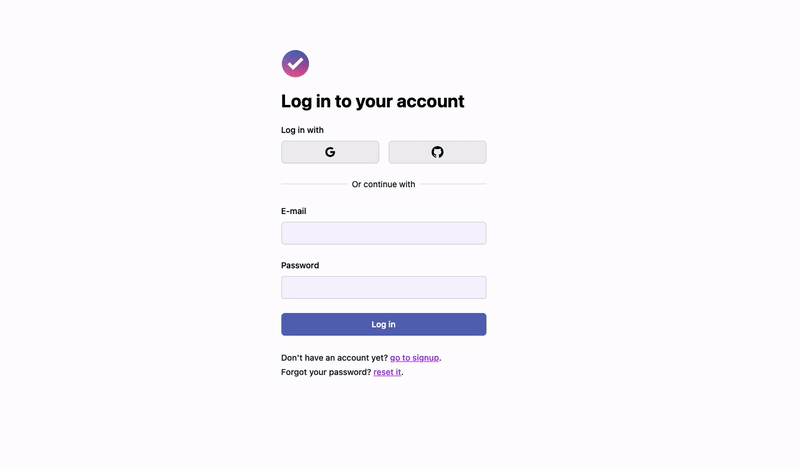 | null | https://wasp-lang.dev/docs/auth/ui | To make using authentication in your app as easy as possible, Wasp generates the server-side code but also the client-side UI for you. It enables you to quickly get the login, signup, password reset and email verification flows in your app. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878eccbb99e13903-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:13:49 GMT | Tue, 23 Apr 2024 15:23:49 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=ypke%2FW2pfLPDcyWQr6b2%2F6RH6xOFm3BlK9eWQXe4RFxUJbug9Uvk4LYRhYaCrcr5H%2F7lbqfop6XkYk6%2Fzh8SMOk3mzgmr%2BRN8wrtBCaT%2BMFP0Be1EbtWWIXRglepniE3"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | deff1826367750d2a6c31fac766e5dd8e7765101 | null | 85FE:20ED2:3C22B46:47437A0:6627D02C | HIT | MISS | cache-iad-kiad7000160-IAD | S1713885229.435263,VS0,VE12 | null | null | en | og:image | https://wasp-lang.dev/docs/auth/ui | og:url | Auth UI | Wasp | og:title | To make using authentication in your app as easy as possible, Wasp generates the server-side code but also the client-side UI for you. It enables you to quickly get the login, signup, password reset and email verification flows in your app. | og:description | Auth UI | Wasp | null | Auth UI
To make using authentication in your app as easy as possible, Wasp generates the server-side code but also the client-side UI for you. It enables you to quickly get the login, signup, password reset and email verification flows in your app.
Below we cover all of the available UI components and how to use them.
Overview
After Wasp generates the UI components for your auth, you can use it as is, or customize it to your liking.
Based on the authentication providers you enabled in your main.wasp file, the Auth UI will show the corresponding UI (form and buttons). For example, if you enabled e-mail authentication:
JavaScript
TypeScript
main.wasp
app MyApp {
//...
auth: {
methods: {
email: {},
},
// ...
}
}
You'll get the following UI:
And then if you enable Google and Github:
JavaScript
TypeScript
main.wasp
app MyApp {
//...
auth: {
methods: {
email: {},
google: {},
github: {},
},
// ...
}
}
The form will automatically update to look like this:
Let's go through all of the available components and how to use them.
Auth Components
The following components are available for you to use in your app:
Login form
Signup form
Forgot password form
Reset password form
Verify email form
Login Form
Used with Username & Password, Email, Github, Google and Keycloak authentication.
You can use the LoginForm component to build your login page:
JavaScript
TypeScript
main.wasp
// ...
route LoginRoute { path: "/login", to: LoginPage }
page LoginPage {
component: import { LoginPage } from "@src/LoginPage.jsx"
}
src/LoginPage.jsx
import { LoginForm } from 'wasp/client/auth'
// Use it like this
export function LoginPage() {
return <LoginForm />
}
It will automatically show the correct authentication providers based on your main.wasp file.
Signup Form
Used with Username & Password, Email, Github, Google and Keycloak authentication.
You can use the SignupForm component to build your signup page:
JavaScript
TypeScript
main.wasp
// ...
route SignupRoute { path: "/signup", to: SignupPage }
page SignupPage {
component: import { SignupPage } from "@src/SignupPage.jsx"
}
src/SignupPage.jsx
import { SignupForm } from 'wasp/client/auth'
// Use it like this
export function SignupPage() {
return <SignupForm />
}
It will automatically show the correct authentication providers based on your main.wasp file.
Read more about customizing the signup process like adding additional fields or extra UI in the Auth Overview section.
Forgot Password Form
Used with Email authentication.
If users forget their password, they can use this form to reset it.
You can use the ForgotPasswordForm component to build your own forgot password page:
JavaScript
TypeScript
main.wasp
// ...
route RequestPasswordResetRoute { path: "/request-password-reset", to: RequestPasswordResetPage }
page RequestPasswordResetPage {
component: import { ForgotPasswordPage } from "@src/ForgotPasswordPage.jsx"
}
src/ForgotPasswordPage.jsx
import { ForgotPasswordForm } from 'wasp/client/auth'
// Use it like this
export function ForgotPasswordPage() {
return <ForgotPasswordForm />
}
Reset Password Form
Used with Email authentication.
After users click on the link in the email they receive after submitting the forgot password form, they will be redirected to this form where they can reset their password.
You can use the ResetPasswordForm component to build your reset password page:
JavaScript
TypeScript
main.wasp
// ...
route PasswordResetRoute { path: "/password-reset", to: PasswordResetPage }
page PasswordResetPage {
component: import { ResetPasswordPage } from "@src/ResetPasswordPage.jsx"
}
src/ResetPasswordPage.jsx
import { ResetPasswordForm } from 'wasp/client/auth'
// Use it like this
export function ResetPasswordPage() {
return <ResetPasswordForm />
}
Verify Email Form
Used with Email authentication.
After users sign up, they will receive an email with a link to this form where they can verify their email.
You can use the VerifyEmailForm component to build your email verification page:
JavaScript
TypeScript
main.wasp
// ...
route EmailVerificationRoute { path: "/email-verification", to: EmailVerificationPage }
page EmailVerificationPage {
component: import { VerifyEmailPage } from "@src/VerifyEmailPage.jsx"
}
src/VerifyEmailPage.jsx
import { VerifyEmailForm } from 'wasp/client/auth'
// Use it like this
export function VerifyEmailPage() {
return <VerifyEmailForm />
}
Customization 💅🏻
You customize all of the available forms by passing props to them.
Props you can pass to all of the forms:
appearance - customize the form colors (via design tokens)
logo - path to your logo
socialLayout - layout of the social buttons, which can be vertical or horizontal
1. Customizing the Colors
We use Stitches to style the Auth UI. You can customize the styles by overriding the default theme tokens.
JavaScript
TypeScript
src/appearance.js
export const authAppearance = {
colors: {
brand: '#5969b8', // blue
brandAccent: '#de5998', // pink
submitButtonText: 'white',
},
}
src/LoginPage.jsx
import { LoginForm } from 'wasp/client/auth'
import { authAppearance } from './appearance'
export function LoginPage() {
return (
<LoginForm
// Pass the appearance object to the form
appearance={authAppearance}
/>
)
}
We recommend defining your appearance in a separate file and importing it into your components.
2. Using Your Logo
You can add your logo to the Auth UI by passing the logo prop to any of the components.
JavaScript
TypeScript
src/LoginPage.jsx
import { LoginForm } from 'wasp/client/auth'
import Logo from './logo.png'
export function LoginPage() {
return (
<LoginForm
// Pass in the path to your logo
logo={Logo}
/>
)
}
You can change the layout of the social buttons by passing the socialLayout prop to any of the components. It can be either vertical or horizontal (default).
If we pass in vertical:
JavaScript
TypeScript
src/LoginPage.jsx
import { LoginForm } from 'wasp/client/auth'
export function LoginPage() {
return (
<LoginForm
// Pass in the socialLayout prop
socialLayout="vertical"
/>
)
}
We get this:
Let's Put Everything Together 🪄
If we provide the logo and custom colors:
JavaScript
TypeScript
src/appearance.js
export const appearance = {
colors: {
brand: '#5969b8', // blue
brandAccent: '#de5998', // pink
submitButtonText: 'white',
},
}
src/LoginPage.jsx
import { LoginForm } from 'wasp/client/auth'
import { authAppearance } from './appearance'
import todoLogo from './todoLogo.png'
export function LoginPage() {
return <LoginForm appearance={appearance} logo={todoLogo} />
}
We get a form looking like this: | https://wasp-lang.dev/docs/auth/ui |
|
1 | null | 2024-04-23T15:13:50.528Z | https://wasp-lang.dev/docs/auth/username-and-pass | https://wasp-lang.dev/docs | http | ## Username & Password
Wasp supports username & password authentication out of the box with login and signup flows. It provides you with the server-side implementation and the UI components for the client-side.
## Setting Up Username & Password Authentication[](#setting-up-username--password-authentication "Direct link to Setting Up Username & Password Authentication")
To set up username authentication we need to:
1. Enable username authentication in the Wasp file
2. Add the `User` entity
3. Add the auth routes and pages
4. Use Auth UI components in our pages
Structure of the `main.wasp` file we will end up with:
main.wasp
```
// Configuring e-mail authenticationapp myApp { auth: { ... }}// Defining User entityentity User { ... }// Defining routes and pagesroute SignupRoute { ... }page SignupPage { ... }// ...
```
### 1\. Enable Username Authentication[](#1-enable-username-authentication "Direct link to 1. Enable Username Authentication")
Let's start with adding the following to our `main.wasp` file:
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { // 1. Specify the user entity (we'll define it next) userEntity: User, methods: { // 2. Enable username authentication usernameAndPassword: {}, }, onAuthFailedRedirectTo: "/login" }}
```
Read more about the `usernameAndPassword` auth method options [here](#fields-in-the-usernameandpassword-dict).
### 2\. Add the User Entity[](#2-add-the-user-entity "Direct link to 2. Add the User Entity")
The `User` entity can be as simple as including only the `id` field:
* JavaScript
* TypeScript
main.wasp
```
// 3. Define the user entityentity User {=psl id Int @id @default(autoincrement()) // Add your own fields below // ...psl=}
```
You can read more about how the `User` entity is connected to the rest of the auth system in the [Auth Entities](https://wasp-lang.dev/docs/auth/entities) section of the docs.
### 3\. Add the Routes and Pages[](#3-add-the-routes-and-pages "Direct link to 3. Add the Routes and Pages")
Next, we need to define the routes and pages for the authentication pages.
Add the following to the `main.wasp` file:
* JavaScript
* TypeScript
main.wasp
```
// ...// 4. Define the routesroute LoginRoute { path: "/login", to: LoginPage }page LoginPage { component: import { Login } from "@src/pages/auth.jsx"}route SignupRoute { path: "/signup", to: SignupPage }page SignupPage { component: import { Signup } from "@src/pages/auth.jsx"}
```
We'll define the React components for these pages in the `src/pages/auth.tsx` file below.
### 4\. Create the Client Pages[](#4-create-the-client-pages "Direct link to 4. Create the Client Pages")
info
We are using [Tailwind CSS](https://tailwindcss.com/) to style the pages. Read more about how to add it [here](https://wasp-lang.dev/docs/project/css-frameworks).
Let's create a `auth.tsx` file in the `src/pages` folder and add the following to it:
* JavaScript
* TypeScript
src/pages/auth.jsx
```
import { LoginForm, SignupForm } from 'wasp/client/auth'import { Link } from 'react-router-dom'export function Login() { return ( <Layout> <LoginForm /> <br /> <span className="text-sm font-medium text-gray-900"> Don't have an account yet? <Link to="/signup">go to signup</Link>. </span> </Layout> );}export function Signup() { return ( <Layout> <SignupForm /> <br /> <span className="text-sm font-medium text-gray-900"> I already have an account (<Link to="/login">go to login</Link>). </span> </Layout> );}// A layout component to center the contentexport function Layout({ children }) { return ( <div className="w-full h-full bg-white"> <div className="min-w-full min-h-[75vh] flex items-center justify-center"> <div className="w-full h-full max-w-sm p-5 bg-white"> <div>{children}</div> </div> </div> </div> );}
```
We imported the generated Auth UI components and used them in our pages. Read more about the Auth UI components [here](https://wasp-lang.dev/docs/auth/ui).
### Conclusion[](#conclusion "Direct link to Conclusion")
That's it! We have set up username authentication in our app. 🎉
Running `wasp db migrate-dev` and then `wasp start` should give you a working app with username authentication. If you want to put some of the pages behind authentication, read the [auth overview docs](https://wasp-lang.dev/docs/auth/overview).
Using multiple auth identities for a single user
Wasp currently doesn't support multiple auth identities for a single user. This means, for example, that a user can't have both an email-based auth identity and a Google-based auth identity. This is something we will add in the future with the introduction of the [account merging feature](https://github.com/wasp-lang/wasp/issues/954).
Account merging means that multiple auth identities can be merged into a single user account. For example, a user's email and Google identity can be merged into a single user account. Then the user can log in with either their email or Google account and they will be logged into the same account.
## Customizing the Auth Flow[](#customizing-the-auth-flow "Direct link to Customizing the Auth Flow")
The login and signup flows are pretty standard: they allow the user to sign up and then log in with their username and password. The signup flow validates the username and password and then creates a new user entity in the database.
Read more about the default username and password validation rules in the [auth overview docs](https://wasp-lang.dev/docs/auth/overview#default-validations).
If you require more control in your authentication flow, you can achieve that in the following ways:
1. Create your UI and use `signup` and `login` actions.
2. Create your custom sign-up action which uses the lower-level API, along with your custom code.
### 1\. Using the `signup` and `login` actions[](#1-using-the-signup-and-login-actions "Direct link to 1-using-the-signup-and-login-actions")
#### `login()`[](#login "Direct link to login")
An action for logging in the user.
It takes two arguments:
* `username: string` required
Username of the user logging in.
* `password: string` required
Password of the user logging in.
You can use it like this:
* JavaScript
* TypeScript
src/pages/auth.jsx
```
import { login } from 'wasp/client/auth'import { useState } from 'react'import { useHistory, Link } from 'react-router-dom'export function LoginPage() { const [username, setUsername] = useState('') const [password, setPassword] = useState('') const [error, setError] = useState(null) const history = useHistory() async function handleSubmit(event) { event.preventDefault() try { await login(username, password) history.push('/') } catch (error) { setError(error) } } return ( <form onSubmit={handleSubmit}> {/* ... */} </form> );}
```
note
When using the exposed `login()` function, make sure to implement your redirect on success login logic (e.g. redirecting to home).
#### `signup()`[](#signup "Direct link to signup")
An action for signing up the user. This action does not log in the user, you still need to call `login()`.
It takes one argument:
* `userFields: object` required
It has the following fields:
* `username: string` required
* `password: string` required
info
By default, Wasp will only save the `username` and `password` fields. If you want to add extra fields to your signup process, read about [defining extra signup fields](https://wasp-lang.dev/docs/auth/overview#customizing-the-signup-process).
You can use it like this:
* JavaScript
* TypeScript
src/pages/auth.jsx
```
import { signup, login } from 'wasp/client/auth'import { useState } from 'react'import { useHistory } from 'react-router-dom'import { Link } from 'react-router-dom'export function Signup() { const [username, setUsername] = useState('') const [password, setPassword] = useState('') const [error, setError] = useState(null) const history = useHistory() async function handleSubmit(event) { event.preventDefault() try { await signup({ username, password, }) await login(username, password) history.push("/") } catch (error) { setError(error) } } return ( <form onSubmit={handleSubmit}> {/* ... */} </form> );}
```
### 2\. Creating your custom sign-up action[](#2-creating-your-custom-sign-up-action "Direct link to 2. Creating your custom sign-up action")
The code of your custom sign-up action can look like this:
* JavaScript
* TypeScript
main.wasp
```
// ...action customSignup { fn: import { signup } from "@src/auth/signup.js",}
```
src/auth/signup.js
```
import { ensurePasswordIsPresent, ensureValidPassword, ensureValidUsername, createProviderId, sanitizeAndSerializeProviderData, createUser,} from 'wasp/server/auth'export const signup = async (args, _context) => { ensureValidUsername(args) ensurePasswordIsPresent(args) ensureValidPassword(args) try { const providerId = createProviderId('username', args.username) const providerData = await sanitizeAndSerializeProviderData({ hashedPassword: args.password, }) await createUser( providerId, providerData, // Any additional data you want to store on the User entity {}, ) } catch (e) { return { success: false, message: e.message, } } // Your custom code after sign-up. // ... return { success: true, message: 'User created successfully', }}
```
We suggest using the built-in field validators for your authentication flow. You can import them from `wasp/server/auth`. These are the same validators that Wasp uses internally for the default authentication flow.
#### Username[](#username "Direct link to Username")
* `ensureValidUsername(args)`
Checks if the username is valid and throws an error if it's not. Read more about the validation rules [here](https://wasp-lang.dev/docs/auth/overview#default-validations).
#### Password[](#password "Direct link to Password")
* `ensurePasswordIsPresent(args)`
Checks if the password is present and throws an error if it's not.
* `ensureValidPassword(args)`
Checks if the password is valid and throws an error if it's not. Read more about the validation rules [here](https://wasp-lang.dev/docs/auth/overview#default-validations).
## Using Auth[](#using-auth "Direct link to Using Auth")
To read more about how to set up the logout button and how to get access to the logged-in user in our client and server code, read the [auth overview docs](https://wasp-lang.dev/docs/auth/overview).
### `getUsername`[](#getusername "Direct link to getusername")
If you are looking to access the user's username in your code, you can do that by accessing the info about the user that is stored in the `user.auth.identities` array.
To make things a bit easier for you, Wasp offers the `getUsername` helper.
The `getUsername` helper returns the user's username or `null` if the user doesn't have a username auth identity.
* JavaScript
* TypeScript
src/MainPage.jsx
```
import { getUsername } from 'wasp/auth'const MainPage = ({ user }) => { const username = getUsername(user) // ...}
```
src/tasks.js
```
import { getUsername } from 'wasp/auth'export const createTask = async (args, context) => { const username = getUsername(context.user) // ...}
```
## API Reference[](#api-reference "Direct link to API Reference")
### `userEntity` fields[](#userentity-fields "Direct link to userentity-fields")
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { usernameAndPassword: {}, }, onAuthFailedRedirectTo: "/login" }}entity User {=psl id Int @id @default(autoincrement())psl=}
```
The user entity needs to have the following fields:
* `id` required
It can be of any type, but it needs to be marked with `@id`
You can add any other fields you want to the user entity. Make sure to also define them in the `userSignupFields` field if they need to be set during the sign-up process.
### Fields in the `usernameAndPassword` dict[](#fields-in-the-usernameandpassword-dict "Direct link to fields-in-the-usernameandpassword-dict")
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { usernameAndPassword: { userSignupFields: import { userSignupFields } from "@src/auth/email.js", }, }, onAuthFailedRedirectTo: "/login" }}// ...
```
#### `userSignupFields: ExtImport`[](#usersignupfields-extimport "Direct link to usersignupfields-extimport")
`userSignupFields` defines all the extra fields that need to be set on the `User` during the sign-up process. For example, if you have `address` and `phone` fields on your `User` entity, you can set them by defining the `userSignupFields` like this:
* JavaScript
* TypeScript
src/auth.js
```
import { defineUserSignupFields } from 'wasp/server/auth'export const userSignupFields = defineUserSignupFields({ address: (data) => { if (!data.address) { throw new Error('Address is required') } return data.address } phone: (data) => data.phone,})
```
Read more about the \`userSignupFields\` function \[here\](./overview#1-defining-extra-fields). | null | https://wasp-lang.dev/docs/auth/username-and-pass | Wasp supports username & password authentication out of the box with login and signup flows. It provides you with the server-side implementation and the UI components for the client-side. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878eccc1c9cb3931-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:13:50 GMT | Tue, 23 Apr 2024 15:23:50 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=zCbajsEfLFBdOxb0toHYWAkgHUZqYe0aBsF%2BikDBKo83S3lOc6cjyy8X8JsExBL6MkdouTcLxvtBgpLBtHr3MTDQSj%2BKFDRGv6nMkEbEgIQ3PMb2Adm77DCEkIcvA2hw"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 19b6980ee752644a4c3c36d5942decccad0f78ee | null | C444:53E77:3A81190:459D74E:6627D02D | HIT | MISS | cache-iad-kiad7000073-IAD | S1713885230.394492,VS0,VE16 | null | null | en | og:image | https://wasp-lang.dev/docs/auth/username-and-pass | og:url | Username & Password | Wasp | og:title | Wasp supports username & password authentication out of the box with login and signup flows. It provides you with the server-side implementation and the UI components for the client-side. | og:description | Username & Password | Wasp | null | Username & Password
Wasp supports username & password authentication out of the box with login and signup flows. It provides you with the server-side implementation and the UI components for the client-side.
Setting Up Username & Password Authentication
To set up username authentication we need to:
Enable username authentication in the Wasp file
Add the User entity
Add the auth routes and pages
Use Auth UI components in our pages
Structure of the main.wasp file we will end up with:
main.wasp
// Configuring e-mail authentication
app myApp {
auth: { ... }
}
// Defining User entity
entity User { ... }
// Defining routes and pages
route SignupRoute { ... }
page SignupPage { ... }
// ...
1. Enable Username Authentication
Let's start with adding the following to our main.wasp file:
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
// 1. Specify the user entity (we'll define it next)
userEntity: User,
methods: {
// 2. Enable username authentication
usernameAndPassword: {},
},
onAuthFailedRedirectTo: "/login"
}
}
Read more about the usernameAndPassword auth method options here.
2. Add the User Entity
The User entity can be as simple as including only the id field:
JavaScript
TypeScript
main.wasp
// 3. Define the user entity
entity User {=psl
id Int @id @default(autoincrement())
// Add your own fields below
// ...
psl=}
You can read more about how the User entity is connected to the rest of the auth system in the Auth Entities section of the docs.
3. Add the Routes and Pages
Next, we need to define the routes and pages for the authentication pages.
Add the following to the main.wasp file:
JavaScript
TypeScript
main.wasp
// ...
// 4. Define the routes
route LoginRoute { path: "/login", to: LoginPage }
page LoginPage {
component: import { Login } from "@src/pages/auth.jsx"
}
route SignupRoute { path: "/signup", to: SignupPage }
page SignupPage {
component: import { Signup } from "@src/pages/auth.jsx"
}
We'll define the React components for these pages in the src/pages/auth.tsx file below.
4. Create the Client Pages
info
We are using Tailwind CSS to style the pages. Read more about how to add it here.
Let's create a auth.tsx file in the src/pages folder and add the following to it:
JavaScript
TypeScript
src/pages/auth.jsx
import { LoginForm, SignupForm } from 'wasp/client/auth'
import { Link } from 'react-router-dom'
export function Login() {
return (
<Layout>
<LoginForm />
<br />
<span className="text-sm font-medium text-gray-900">
Don't have an account yet? <Link to="/signup">go to signup</Link>.
</span>
</Layout>
);
}
export function Signup() {
return (
<Layout>
<SignupForm />
<br />
<span className="text-sm font-medium text-gray-900">
I already have an account (<Link to="/login">go to login</Link>).
</span>
</Layout>
);
}
// A layout component to center the content
export function Layout({ children }) {
return (
<div className="w-full h-full bg-white">
<div className="min-w-full min-h-[75vh] flex items-center justify-center">
<div className="w-full h-full max-w-sm p-5 bg-white">
<div>{children}</div>
</div>
</div>
</div>
);
}
We imported the generated Auth UI components and used them in our pages. Read more about the Auth UI components here.
Conclusion
That's it! We have set up username authentication in our app. 🎉
Running wasp db migrate-dev and then wasp start should give you a working app with username authentication. If you want to put some of the pages behind authentication, read the auth overview docs.
Using multiple auth identities for a single user
Wasp currently doesn't support multiple auth identities for a single user. This means, for example, that a user can't have both an email-based auth identity and a Google-based auth identity. This is something we will add in the future with the introduction of the account merging feature.
Account merging means that multiple auth identities can be merged into a single user account. For example, a user's email and Google identity can be merged into a single user account. Then the user can log in with either their email or Google account and they will be logged into the same account.
Customizing the Auth Flow
The login and signup flows are pretty standard: they allow the user to sign up and then log in with their username and password. The signup flow validates the username and password and then creates a new user entity in the database.
Read more about the default username and password validation rules in the auth overview docs.
If you require more control in your authentication flow, you can achieve that in the following ways:
Create your UI and use signup and login actions.
Create your custom sign-up action which uses the lower-level API, along with your custom code.
1. Using the signup and login actions
login()
An action for logging in the user.
It takes two arguments:
username: string required
Username of the user logging in.
password: string required
Password of the user logging in.
You can use it like this:
JavaScript
TypeScript
src/pages/auth.jsx
import { login } from 'wasp/client/auth'
import { useState } from 'react'
import { useHistory, Link } from 'react-router-dom'
export function LoginPage() {
const [username, setUsername] = useState('')
const [password, setPassword] = useState('')
const [error, setError] = useState(null)
const history = useHistory()
async function handleSubmit(event) {
event.preventDefault()
try {
await login(username, password)
history.push('/')
} catch (error) {
setError(error)
}
}
return (
<form onSubmit={handleSubmit}>
{/* ... */}
</form>
);
}
note
When using the exposed login() function, make sure to implement your redirect on success login logic (e.g. redirecting to home).
signup()
An action for signing up the user. This action does not log in the user, you still need to call login().
It takes one argument:
userFields: object required
It has the following fields:
username: string required
password: string required
info
By default, Wasp will only save the username and password fields. If you want to add extra fields to your signup process, read about defining extra signup fields.
You can use it like this:
JavaScript
TypeScript
src/pages/auth.jsx
import { signup, login } from 'wasp/client/auth'
import { useState } from 'react'
import { useHistory } from 'react-router-dom'
import { Link } from 'react-router-dom'
export function Signup() {
const [username, setUsername] = useState('')
const [password, setPassword] = useState('')
const [error, setError] = useState(null)
const history = useHistory()
async function handleSubmit(event) {
event.preventDefault()
try {
await signup({
username,
password,
})
await login(username, password)
history.push("/")
} catch (error) {
setError(error)
}
}
return (
<form onSubmit={handleSubmit}>
{/* ... */}
</form>
);
}
2. Creating your custom sign-up action
The code of your custom sign-up action can look like this:
JavaScript
TypeScript
main.wasp
// ...
action customSignup {
fn: import { signup } from "@src/auth/signup.js",
}
src/auth/signup.js
import {
ensurePasswordIsPresent,
ensureValidPassword,
ensureValidUsername,
createProviderId,
sanitizeAndSerializeProviderData,
createUser,
} from 'wasp/server/auth'
export const signup = async (args, _context) => {
ensureValidUsername(args)
ensurePasswordIsPresent(args)
ensureValidPassword(args)
try {
const providerId = createProviderId('username', args.username)
const providerData = await sanitizeAndSerializeProviderData({
hashedPassword: args.password,
})
await createUser(
providerId,
providerData,
// Any additional data you want to store on the User entity
{},
)
} catch (e) {
return {
success: false,
message: e.message,
}
}
// Your custom code after sign-up.
// ...
return {
success: true,
message: 'User created successfully',
}
}
We suggest using the built-in field validators for your authentication flow. You can import them from wasp/server/auth. These are the same validators that Wasp uses internally for the default authentication flow.
Username
ensureValidUsername(args)
Checks if the username is valid and throws an error if it's not. Read more about the validation rules here.
Password
ensurePasswordIsPresent(args)
Checks if the password is present and throws an error if it's not.
ensureValidPassword(args)
Checks if the password is valid and throws an error if it's not. Read more about the validation rules here.
Using Auth
To read more about how to set up the logout button and how to get access to the logged-in user in our client and server code, read the auth overview docs.
getUsername
If you are looking to access the user's username in your code, you can do that by accessing the info about the user that is stored in the user.auth.identities array.
To make things a bit easier for you, Wasp offers the getUsername helper.
The getUsername helper returns the user's username or null if the user doesn't have a username auth identity.
JavaScript
TypeScript
src/MainPage.jsx
import { getUsername } from 'wasp/auth'
const MainPage = ({ user }) => {
const username = getUsername(user)
// ...
}
src/tasks.js
import { getUsername } from 'wasp/auth'
export const createTask = async (args, context) => {
const username = getUsername(context.user)
// ...
}
API Reference
userEntity fields
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
usernameAndPassword: {},
},
onAuthFailedRedirectTo: "/login"
}
}
entity User {=psl
id Int @id @default(autoincrement())
psl=}
The user entity needs to have the following fields:
id required
It can be of any type, but it needs to be marked with @id
You can add any other fields you want to the user entity. Make sure to also define them in the userSignupFields field if they need to be set during the sign-up process.
Fields in the usernameAndPassword dict
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
usernameAndPassword: {
userSignupFields: import { userSignupFields } from "@src/auth/email.js",
},
},
onAuthFailedRedirectTo: "/login"
}
}
// ...
userSignupFields: ExtImport
userSignupFields defines all the extra fields that need to be set on the User during the sign-up process. For example, if you have address and phone fields on your User entity, you can set them by defining the userSignupFields like this:
JavaScript
TypeScript
src/auth.js
import { defineUserSignupFields } from 'wasp/server/auth'
export const userSignupFields = defineUserSignupFields({
address: (data) => {
if (!data.address) {
throw new Error('Address is required')
}
return data.address
}
phone: (data) => data.phone,
})
Read more about the `userSignupFields` function [here](./overview#1-defining-extra-fields). | https://wasp-lang.dev/docs/auth/username-and-pass |
|
1 | 200 | 2024-04-23T15:13:47.752Z | https://wasp-lang.dev/docs/auth/overview | https://wasp-lang.dev/docs | browser | ## Overview
Auth is an essential piece of any serious application. That's why Wasp provides authentication and authorization support out of the box.
Here's a 1-minute tour of how full-stack auth works in Wasp:
Enabling auth for your app is optional and can be done by configuring the `auth` field of your `app` declaration:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { title: "My app", //... auth: { userEntity: User, methods: { usernameAndPassword: {}, // use this or email, not both email: {}, // use this or usernameAndPassword, not both google: {}, gitHub: {}, }, onAuthFailedRedirectTo: "/someRoute" }}//...
```
Read more about the `auth` field options in the [API Reference](#api-reference) section.
We will provide a quick overview of auth in Wasp and link to more detailed documentation for each auth method.
## Available auth methods[](#available-auth-methods "Direct link to Available auth methods")
Wasp supports the following auth methods:
Click on each auth method for more details.
Let's say we enabled the [Username & password](https://wasp-lang.dev/docs/auth/username-and-pass) authentication.
We get an auth backend with signup and login endpoints. We also get the `user` object in our [Operations](https://wasp-lang.dev/docs/data-model/operations/overview) and we can decide what to do based on whether the user is logged in or not.
We would also get the [Auth UI](https://wasp-lang.dev/docs/auth/ui) generated for us. We can set up our login and signup pages where our users can **create their account** and **login**. We can then protect certain pages by setting `authRequired: true` for them. This will make sure that only logged-in users can access them.
We will also have access to the `user` object in our frontend code, so we can show different UI to logged-in and logged-out users. For example, we can show the user's name in the header alongside a **logout button** or a login button if the user is not logged in.
## Protecting a page with `authRequired`[](#protecting-a-page-with-authrequired "Direct link to protecting-a-page-with-authrequired")
When declaring a page, you can set the `authRequired` property.
If you set it to `true`, only authenticated users can access the page. Unauthenticated users are redirected to a route defined by the `app.auth.onAuthFailedRedirectTo` field.
* JavaScript
* TypeScript
main.wasp
```
page MainPage { component: import Main from "@src/pages/Main", authRequired: true}
```
Requires auth method
You can only use `authRequired` if your app uses one of the [available auth methods](#available-auth-methods).
If `authRequired` is set to `true`, the page's React component (specified by the `component` property) receives the `user` object as a prop. Read more about the `user` object in the [Accessing the logged-in user section](#accessing-the-logged-in-user).
## Logout action[](#logout-action "Direct link to Logout action")
We provide an action for logging out the user. Here's how you can use it:
* JavaScript
* TypeScript
src/components/LogoutButton.jsx
```
import { logout } from 'wasp/client/auth'const LogoutButton = () => { return <button onClick={logout}>Logout</button>}
```
## Accessing the logged-in user[](#accessing-the-logged-in-user "Direct link to Accessing the logged-in user")
You can get access to the `user` object both on the server and on the client. The `user` object contains the logged-in user's data.
The `user` object has all the fields that you defined in your `User` entity, plus the `auth` field which contains the auth identities connected to the user. For example, if the user signed up with their email, the `user` object might look something like this:
```
const user = { id: "19c7d164-b5cb-4dde-a0cc-0daea77cf854", // Your entity's fields. address: "My address", // ... // Auth identities connected to the user. auth: { id: "26ab6f96-ed76-4ee5-9ac3-2fd0bf19711f", identities: [ { providerName: "email", providerUserId: "some@email.com", providerData: { ... }, }, ] },}
```
You can read more about how the `User` entity is connected to the rest of the auth system in the [Auth Entities](https://wasp-lang.dev/docs/auth/entities) section of the docs.
### On the client[](#on-the-client "Direct link to On the client")
There are two ways to access the `user` object on the client:
* the `user` prop
* the `useAuth` hook
#### Using the `user` prop[](#using-the-user-prop "Direct link to using-the-user-prop")
If the page's declaration sets `authRequired` to `true`, the page's React component receives the `user` object as a prop:
* JavaScript
* TypeScript
main.wasp
```
// ...page AccountPage { component: import Account from "@src/pages/Account", authRequired: true}
```
src/pages/Account.jsx
```
import Button from './Button'import { logout } from 'wasp/client/auth'const AccountPage = ({ user }) => { return ( <div> <Button onClick={logout}>Logout</Button> {JSON.stringify(user, null, 2)} </div> )}export default AccountPage
```
#### Using the `useAuth` hook[](#using-the-useauth-hook "Direct link to using-the-useauth-hook")
Wasp provides a React hook you can use in the client components - `useAuth`.
This hook is a thin wrapper over Wasp's `useQuery` hook and returns data in the same format.
* JavaScript
* TypeScript
src/pages/MainPage.jsx
```
import { useAuth, logout } from 'wasp/client/auth'import { Link } from 'react-router-dom'import Todo from '../Todo'export function Main() { const { data: user } = useAuth() if (!user) { return ( <span> Please <Link to="/login">login</Link> or{' '} <Link to="/signup">sign up</Link>. </span> ) } else { return ( <> <button onClick={logout}>Logout</button> <Todo /> </> ) }}
```
tip
Since the `user` prop is only available in a page's React component: use the `user` prop in the page's React component and the `useAuth` hook in any other React component.
### On the server[](#on-the-server "Direct link to On the server")
#### Using the `context.user` object[](#using-the-contextuser-object "Direct link to using-the-contextuser-object")
When authentication is enabled, all [queries and actions](https://wasp-lang.dev/docs/data-model/operations/overview) have access to the `user` object through the `context` argument. `context.user` contains all User entity's fields and the auth identities connected to the user. We strip out the `hashedPassword` field from the identities for security reasons.
* JavaScript
* TypeScript
src/actions.js
```
import { HttpError } from 'wasp/server'export const createTask = async (task, context) => { if (!context.user) { throw new HttpError(403) } const Task = context.entities.Task return Task.create({ data: { description: task.description, user: { connect: { id: context.user.id }, }, }, })}
```
To implement access control in your app, each operation must check `context.user` and decide what to do. For example, if `context.user` is `undefined` inside a private operation, the user's access should be denied.
When using WebSockets, the `user` object is also available on the `socket.data` object. Read more in the [WebSockets section](https://wasp-lang.dev/docs/advanced/web-sockets#websocketfn-function).
## Sessions[](#sessions "Direct link to Sessions")
Wasp's auth uses sessions to keep track of the logged-in user. The session is stored in `localStorage` on the client and in the database on the server. Under the hood, Wasp uses the excellent [Lucia Auth v3](https://v3.lucia-auth.com/) library for session management.
When users log in, Wasp creates a session for them and stores it in the database. The session is then sent to the client and stored in `localStorage`. When users log out, Wasp deletes the session from the database and from `localStorage`.
## User Entity[](#user-entity "Direct link to User Entity")
### Password Hashing[](#password-hashing "Direct link to Password Hashing")
If you are saving a user's password in the database, you should **never** save it as plain text. You can use Wasp's helper functions for serializing and deserializing provider data which will automatically hash the password for you:
main.wasp
```
// ...action updatePassword { fn: import { updatePassword } from "@src/auth",}
```
* JavaScript
* TypeScript
src/auth.js
```
import { createProviderId, findAuthIdentity, updateAuthIdentityProviderData, deserializeAndSanitizeProviderData,} from 'wasp/server/auth';export const updatePassword = async (args, context) => { const providerId = createProviderId('email', args.email) const authIdentity = await findAuthIdentity(providerId) if (!authIdentity) { throw new HttpError(400, "Unknown user") } const providerData = deserializeAndSanitizeProviderData(authIdentity.providerData) // Updates the password and hashes it automatically. await updateAuthIdentityProviderData(providerId, providerData, { hashedPassword: args.password, })}
```
### Default Validations[](#default-validations "Direct link to Default Validations")
When you are using the default authentication flow, Wasp validates the fields with some default validations. These validations run if you use Wasp's built-in [Auth UI](https://wasp-lang.dev/docs/auth/ui) or if you use the provided auth actions.
If you decide to create your [custom auth actions](https://wasp-lang.dev/docs/auth/username-and-pass#2-creating-your-custom-sign-up-action), you'll need to run the validations yourself.
Default validations depend on the auth method you use.
#### Username & Password[](#username--password "Direct link to Username & Password")
If you use [Username & password](https://wasp-lang.dev/docs/auth/username-and-pass) authentication, the default validations are:
* The `username` must not be empty
* The `password` must not be empty, have at least 8 characters, and contain a number
Note that `username`s are stored in a **case-insensitive** manner.
#### Email[](#email "Direct link to Email")
If you use [Email](https://wasp-lang.dev/docs/auth/email) authentication, the default validations are:
* The `email` must not be empty and a valid email address
* The `password` must not be empty, have at least 8 characters, and contain a number
Note that `email`s are stored in a **case-insensitive** manner.
## Customizing the Signup Process[](#customizing-the-signup-process "Direct link to Customizing the Signup Process")
Sometimes you want to include **extra fields** in your signup process, like first name and last name and save them in the `User` entity.
For this to happen:
* you need to define the fields that you want saved in the database,
* you need to customize the `SignupForm` (in the case of [Email](https://wasp-lang.dev/docs/auth/email) or [Username & Password](https://wasp-lang.dev/docs/auth/username-and-pass) auth)
Other times, you might need to just add some **extra UI** elements to the form, like a checkbox for terms of service. In this case, customizing only the UI components is enough.
Let's see how to do both.
If we want to **save** some extra fields in our signup process, we need to tell our app they exist.
We do that by defining an object where the keys represent the field name, and the values are functions that receive the data sent from the client\* and return the value of the field.
\* We exclude the `password` field from this object to prevent it from being saved as plain-text in the database. The `password` field is handled by Wasp's auth backend.
First, we add the `auth.methods.{authMethod}.userSignupFields` field in our `main.wasp` file. The `{authMethod}` depends on the auth method you are using.
For example, if you are using [Username & Password](https://wasp-lang.dev/docs/auth/username-and-pass), you would add the `auth.methods.usernameAndPassword.userSignupFields` field:
* JavaScript
* TypeScript
main.wasp
```
app crudTesting { // ... auth: { userEntity: User, methods: { usernameAndPassword: { userSignupFields: import { userSignupFields } from "@src/auth/signup", }, }, onAuthFailedRedirectTo: "/login", },}entity User {=psl id Int @id @default(autoincrement()) address String?psl=}
```
Then we'll define the `userSignupFields` object in the `src/auth/signup.js` file:
src/auth/signup.js
```
import { defineUserSignupFields } from 'wasp/server/auth'export const userSignupFields = defineUserSignupFields({ address: async (data) => { const address = data.address if (typeof address !== 'string') { throw new Error('Address is required') } if (address.length < 5) { throw new Error('Address must be at least 5 characters long') } return address },})
```
Read more about the `userSignupFields` object in the [API Reference](#signup-fields-customization).
Keep in mind, that these field names need to exist on the `userEntity` you defined in your `main.wasp` file e.g. `address` needs to be a field on the `User` entity.
The field function will receive the data sent from the client and it needs to return the value that will be saved into the database. If the field is invalid, the function should throw an error.
Using Validation Libraries
You can use any validation library you want to validate the fields. For example, you can use `zod` like this:
Click to see the code
Now that we defined the fields, Wasp knows how to:
1. Validate the data sent from the client
2. Save the data to the database
Next, let's see how to customize [Auth UI](https://wasp-lang.dev/docs/auth/ui) to include those fields.
### 2\. Customizing the Signup Component[](#2-customizing-the-signup-component "Direct link to 2. Customizing the Signup Component")
Using Custom Signup Component
If you are not using Wasp's Auth UI, you can skip this section. Just make sure to include the extra fields in your custom signup form.
Read more about using the signup actions for:
* email auth [here](https://wasp-lang.dev/docs/auth/email#fields-in-the-email-dict)
* username & password auth [here](https://wasp-lang.dev/docs/auth/username-and-pass#customizing-the-auth-flow)
If you are using Wasp's Auth UI, you can customize the `SignupForm` component by passing the `additionalFields` prop to it. It can be either a list of extra fields or a render function.
When you pass in a list of extra fields to the `SignupForm`, they are added to the form one by one, in the order you pass them in.
Inside the list, there can be either **objects** or **render functions** (you can combine them):
1. Objects are a simple way to describe new fields you need, but a bit less flexible than render functions.
2. Render functions can be used to render any UI you want, but they require a bit more code. The render functions receive the `react-hook-form` object and the form state object as arguments.
* JavaScript
* TypeScript
src/SignupPage.jsx
```
import { SignupForm, FormError, FormInput, FormItemGroup, FormLabel,} from 'wasp/client/auth'export const SignupPage = () => { return ( <SignupForm additionalFields={[ /* The address field is defined using an object */ { name: 'address', label: 'Address', type: 'input', validations: { required: 'Address is required', }, }, /* The phone number is defined using a render function */ (form, state) => { return ( <FormItemGroup> <FormLabel>Phone Number</FormLabel> <FormInput {...form.register('phoneNumber', { required: 'Phone number is required', })} disabled={state.isLoading} /> {form.formState.errors.phoneNumber && ( <FormError> {form.formState.errors.phoneNumber.message} </FormError> )} </FormItemGroup> ) }, ]} /> )}
```
Read more about the extra fields in the [API Reference](#signupform-customization).
#### Using a Single Render Function[](#using-a-single-render-function "Direct link to Using a Single Render Function")
Instead of passing in a list of extra fields, you can pass in a render function which will receive the `react-hook-form` object and the form state object as arguments. What ever the render function returns, will be rendered below the default fields.
* JavaScript
* TypeScript
src/SignupPage.jsx
```
import { SignupForm, FormItemGroup } from 'wasp/client/auth'export const SignupPage = () => { return ( <SignupForm additionalFields={(form, state) => { const username = form.watch('username') return ( username && ( <FormItemGroup> Hello there <strong>{username}</strong> 👋 </FormItemGroup> ) ) }} /> )}
```
Read more about the render function in the [API Reference](#signupform-customization).
## API Reference[](#api-reference "Direct link to API Reference")
### Auth Fields[](#auth-fields "Direct link to Auth Fields")
* JavaScript
* TypeScript
main.wasp
```
title: "My app", //... auth: { userEntity: User, methods: { usernameAndPassword: {}, // use this or email, not both email: {}, // use this or usernameAndPassword, not both google: {}, gitHub: {}, }, onAuthFailedRedirectTo: "/someRoute", signup: { ... } }}//...
```
`app.auth` is a dictionary with the following fields:
#### `userEntity: entity` required[](#userentity-entity- "Direct link to userentity-entity-")
The entity representing the user connected to your business logic.
You can read more about how the `User` entity is connected to the rest of the auth system in the [Auth Entities](https://wasp-lang.dev/docs/auth/entities) section of the docs.
#### `methods: dict` required[](#methods-dict- "Direct link to methods-dict-")
A dictionary of auth methods enabled for the app.
Click on each auth method for more details.
#### `onAuthFailedRedirectTo: String` required[](#onauthfailedredirectto-string- "Direct link to onauthfailedredirectto-string-")
The route to which Wasp should redirect unauthenticated user when they try to access a private page (i.e., a page that has `authRequired: true`). Check out these [essentials docs on auth](https://wasp-lang.dev/docs/tutorial/auth#adding-auth-to-the-project) to see an example of usage.
#### `onAuthSucceededRedirectTo: String`[](#onauthsucceededredirectto-string "Direct link to onauthsucceededredirectto-string")
The route to which Wasp will send a successfully authenticated after a successful login/signup. The default value is `"/"`.
note
Automatic redirect on successful login only works when using the Wasp-provided [Auth UI](https://wasp-lang.dev/docs/auth/ui).
#### `signup: SignupOptions`[](#signup-signupoptions "Direct link to signup-signupoptions")
Read more about the signup process customization API in the [Signup Fields Customization](#signup-fields-customization) section.
### Signup Fields Customization[](#signup-fields-customization "Direct link to Signup Fields Customization")
If you want to add extra fields to the signup process, the server needs to know how to save them to the database. You do that by defining the `auth.methods.{authMethod}.userSignupFields` field in your `main.wasp` file.
* JavaScript
* TypeScript
main.wasp
```
app crudTesting { // ... auth: { userEntity: User, methods: { usernameAndPassword: { userSignupFields: import { userSignupFields } from "@src/auth/signup", }, }, onAuthFailedRedirectTo: "/login", },}
```
Then we'll export the `userSignupFields` object from the `src/auth/signup.js` file:
src/auth/signup.js
```
import { defineUserSignupFields } from 'wasp/server/auth'export const userSignupFields = defineUserSignupFields({ address: async (data) => { const address = data.address if (typeof address !== 'string') { throw new Error('Address is required') } if (address.length < 5) { throw new Error('Address must be at least 5 characters long') } return address },})
```
The `userSignupFields` object is an object where the keys represent the field name, and the values are functions that receive the data sent from the client\* and return the value of the field.
If the value that the function received is invalid, the function should throw an error.
\* We exclude the `password` field from this object to prevent it from being saved as plain text in the database. The `password` field is handled by Wasp's auth backend.
### `SignupForm` Customization[](#signupform-customization "Direct link to signupform-customization")
To customize the `SignupForm` component, you need to pass in the `additionalFields` prop. It can be either a list of extra fields or a render function.
* JavaScript
* TypeScript
src/SignupPage.jsx
```
import { SignupForm, FormError, FormInput, FormItemGroup, FormLabel,} from 'wasp/client/auth'export const SignupPage = () => { return ( <SignupForm additionalFields={[ { name: 'address', label: 'Address', type: 'input', validations: { required: 'Address is required', }, }, (form, state) => { return ( <FormItemGroup> <FormLabel>Phone Number</FormLabel> <FormInput {...form.register('phoneNumber', { required: 'Phone number is required', })} disabled={state.isLoading} /> {form.formState.errors.phoneNumber && ( <FormError> {form.formState.errors.phoneNumber.message} </FormError> )} </FormItemGroup> ) }, ]} /> )}
```
The extra fields can be either **objects** or **render functions** (you can combine them):
1. Objects are a simple way to describe new fields you need, but a bit less flexible than render functions.
The objects have the following properties:
* `name` required
* the name of the field
* `label` required
* the label of the field (used in the UI)
* `type` required
* the type of the field, which can be `input` or `textarea`
* `validations`
* an object with the validation rules for the field. The keys are the validation names, and the values are the validation error messages. Read more about the available validation rules in the [react-hook-form docs](https://react-hook-form.com/api/useform/register#register).
2. Render functions receive the `react-hook-form` object and the form state as arguments, and they can use them to render arbitrary UI elements.
The render function has the following signature:
```
;(form: UseFormReturn, state: FormState) => React.ReactNode
```
* `form` required
* the `react-hook-form` object, read more about it in the [react-hook-form docs](https://react-hook-form.com/api/useform)
* you need to use the `form.register` function to register your fields
* `state` required
* the form state object which has the following properties:
* `isLoading: boolean`
* whether the form is currently submitting | null | https://wasp-lang.dev/docs/auth/overview | Auth is an essential piece of any serious application. That's why Wasp provides authentication and authorization support out of the box. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecc883b624bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:13:41 GMT | Tue, 23 Apr 2024 15:23:41 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=N5Xx1Ny4JuiBSfrf7%2B%2Fd7RYvj8s6oeiULh8OLqOJ7MDN3bpkYV9ggubRv52ADbZplJGSKUrZxJhCCNSTTsVnZG0sAloj421Tn%2BFkJrhgwDNKXiL4CU7WkkI600EOntKs"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | cb8f22656b26decda94f61ad3e206190e4ae8f7e | h2 | 83B6:2F9FB7:3A5BBF3:4577AE7:6627D025 | null | MISS | cache-nyc-kteb1890087-NYC | S1713885221.167233, VS0, VE32 | null | null | en | og:image | https://wasp-lang.dev/docs/auth/overview | og:url | Overview | Wasp | og:title | Auth is an essential piece of any serious application. That's why Wasp provides authentication and authorization support out of the box. | og:description | Overview | Wasp | null | Overview
Auth is an essential piece of any serious application. That's why Wasp provides authentication and authorization support out of the box.
Here's a 1-minute tour of how full-stack auth works in Wasp:
Enabling auth for your app is optional and can be done by configuring the auth field of your app declaration:
JavaScript
TypeScript
main.wasp
app MyApp {
title: "My app",
//...
auth: {
userEntity: User,
methods: {
usernameAndPassword: {}, // use this or email, not both
email: {}, // use this or usernameAndPassword, not both
google: {},
gitHub: {},
},
onAuthFailedRedirectTo: "/someRoute"
}
}
//...
Read more about the auth field options in the API Reference section.
We will provide a quick overview of auth in Wasp and link to more detailed documentation for each auth method.
Available auth methods
Wasp supports the following auth methods:
Click on each auth method for more details.
Let's say we enabled the Username & password authentication.
We get an auth backend with signup and login endpoints. We also get the user object in our Operations and we can decide what to do based on whether the user is logged in or not.
We would also get the Auth UI generated for us. We can set up our login and signup pages where our users can create their account and login. We can then protect certain pages by setting authRequired: true for them. This will make sure that only logged-in users can access them.
We will also have access to the user object in our frontend code, so we can show different UI to logged-in and logged-out users. For example, we can show the user's name in the header alongside a logout button or a login button if the user is not logged in.
Protecting a page with authRequired
When declaring a page, you can set the authRequired property.
If you set it to true, only authenticated users can access the page. Unauthenticated users are redirected to a route defined by the app.auth.onAuthFailedRedirectTo field.
JavaScript
TypeScript
main.wasp
page MainPage {
component: import Main from "@src/pages/Main",
authRequired: true
}
Requires auth method
You can only use authRequired if your app uses one of the available auth methods.
If authRequired is set to true, the page's React component (specified by the component property) receives the user object as a prop. Read more about the user object in the Accessing the logged-in user section.
Logout action
We provide an action for logging out the user. Here's how you can use it:
JavaScript
TypeScript
src/components/LogoutButton.jsx
import { logout } from 'wasp/client/auth'
const LogoutButton = () => {
return <button onClick={logout}>Logout</button>
}
Accessing the logged-in user
You can get access to the user object both on the server and on the client. The user object contains the logged-in user's data.
The user object has all the fields that you defined in your User entity, plus the auth field which contains the auth identities connected to the user. For example, if the user signed up with their email, the user object might look something like this:
const user = {
id: "19c7d164-b5cb-4dde-a0cc-0daea77cf854",
// Your entity's fields.
address: "My address",
// ...
// Auth identities connected to the user.
auth: {
id: "26ab6f96-ed76-4ee5-9ac3-2fd0bf19711f",
identities: [
{
providerName: "email",
providerUserId: "some@email.com",
providerData: { ... },
},
]
},
}
You can read more about how the User entity is connected to the rest of the auth system in the Auth Entities section of the docs.
On the client
There are two ways to access the user object on the client:
the user prop
the useAuth hook
Using the user prop
If the page's declaration sets authRequired to true, the page's React component receives the user object as a prop:
JavaScript
TypeScript
main.wasp
// ...
page AccountPage {
component: import Account from "@src/pages/Account",
authRequired: true
}
src/pages/Account.jsx
import Button from './Button'
import { logout } from 'wasp/client/auth'
const AccountPage = ({ user }) => {
return (
<div>
<Button onClick={logout}>Logout</Button>
{JSON.stringify(user, null, 2)}
</div>
)
}
export default AccountPage
Using the useAuth hook
Wasp provides a React hook you can use in the client components - useAuth.
This hook is a thin wrapper over Wasp's useQuery hook and returns data in the same format.
JavaScript
TypeScript
src/pages/MainPage.jsx
import { useAuth, logout } from 'wasp/client/auth'
import { Link } from 'react-router-dom'
import Todo from '../Todo'
export function Main() {
const { data: user } = useAuth()
if (!user) {
return (
<span>
Please <Link to="/login">login</Link> or{' '}
<Link to="/signup">sign up</Link>.
</span>
)
} else {
return (
<>
<button onClick={logout}>Logout</button>
<Todo />
</>
)
}
}
tip
Since the user prop is only available in a page's React component: use the user prop in the page's React component and the useAuth hook in any other React component.
On the server
Using the context.user object
When authentication is enabled, all queries and actions have access to the user object through the context argument. context.user contains all User entity's fields and the auth identities connected to the user. We strip out the hashedPassword field from the identities for security reasons.
JavaScript
TypeScript
src/actions.js
import { HttpError } from 'wasp/server'
export const createTask = async (task, context) => {
if (!context.user) {
throw new HttpError(403)
}
const Task = context.entities.Task
return Task.create({
data: {
description: task.description,
user: {
connect: { id: context.user.id },
},
},
})
}
To implement access control in your app, each operation must check context.user and decide what to do. For example, if context.user is undefined inside a private operation, the user's access should be denied.
When using WebSockets, the user object is also available on the socket.data object. Read more in the WebSockets section.
Sessions
Wasp's auth uses sessions to keep track of the logged-in user. The session is stored in localStorage on the client and in the database on the server. Under the hood, Wasp uses the excellent Lucia Auth v3 library for session management.
When users log in, Wasp creates a session for them and stores it in the database. The session is then sent to the client and stored in localStorage. When users log out, Wasp deletes the session from the database and from localStorage.
User Entity
Password Hashing
If you are saving a user's password in the database, you should never save it as plain text. You can use Wasp's helper functions for serializing and deserializing provider data which will automatically hash the password for you:
main.wasp
// ...
action updatePassword {
fn: import { updatePassword } from "@src/auth",
}
JavaScript
TypeScript
src/auth.js
import {
createProviderId,
findAuthIdentity,
updateAuthIdentityProviderData,
deserializeAndSanitizeProviderData,
} from 'wasp/server/auth';
export const updatePassword = async (args, context) => {
const providerId = createProviderId('email', args.email)
const authIdentity = await findAuthIdentity(providerId)
if (!authIdentity) {
throw new HttpError(400, "Unknown user")
}
const providerData = deserializeAndSanitizeProviderData(authIdentity.providerData)
// Updates the password and hashes it automatically.
await updateAuthIdentityProviderData(providerId, providerData, {
hashedPassword: args.password,
})
}
Default Validations
When you are using the default authentication flow, Wasp validates the fields with some default validations. These validations run if you use Wasp's built-in Auth UI or if you use the provided auth actions.
If you decide to create your custom auth actions, you'll need to run the validations yourself.
Default validations depend on the auth method you use.
Username & Password
If you use Username & password authentication, the default validations are:
The username must not be empty
The password must not be empty, have at least 8 characters, and contain a number
Note that usernames are stored in a case-insensitive manner.
Email
If you use Email authentication, the default validations are:
The email must not be empty and a valid email address
The password must not be empty, have at least 8 characters, and contain a number
Note that emails are stored in a case-insensitive manner.
Customizing the Signup Process
Sometimes you want to include extra fields in your signup process, like first name and last name and save them in the User entity.
For this to happen:
you need to define the fields that you want saved in the database,
you need to customize the SignupForm (in the case of Email or Username & Password auth)
Other times, you might need to just add some extra UI elements to the form, like a checkbox for terms of service. In this case, customizing only the UI components is enough.
Let's see how to do both.
If we want to save some extra fields in our signup process, we need to tell our app they exist.
We do that by defining an object where the keys represent the field name, and the values are functions that receive the data sent from the client* and return the value of the field.
* We exclude the password field from this object to prevent it from being saved as plain-text in the database. The password field is handled by Wasp's auth backend.
First, we add the auth.methods.{authMethod}.userSignupFields field in our main.wasp file. The {authMethod} depends on the auth method you are using.
For example, if you are using Username & Password, you would add the auth.methods.usernameAndPassword.userSignupFields field:
JavaScript
TypeScript
main.wasp
app crudTesting {
// ...
auth: {
userEntity: User,
methods: {
usernameAndPassword: {
userSignupFields: import { userSignupFields } from "@src/auth/signup",
},
},
onAuthFailedRedirectTo: "/login",
},
}
entity User {=psl
id Int @id @default(autoincrement())
address String?
psl=}
Then we'll define the userSignupFields object in the src/auth/signup.js file:
src/auth/signup.js
import { defineUserSignupFields } from 'wasp/server/auth'
export const userSignupFields = defineUserSignupFields({
address: async (data) => {
const address = data.address
if (typeof address !== 'string') {
throw new Error('Address is required')
}
if (address.length < 5) {
throw new Error('Address must be at least 5 characters long')
}
return address
},
})
Read more about the userSignupFields object in the API Reference.
Keep in mind, that these field names need to exist on the userEntity you defined in your main.wasp file e.g. address needs to be a field on the User entity.
The field function will receive the data sent from the client and it needs to return the value that will be saved into the database. If the field is invalid, the function should throw an error.
Using Validation Libraries
You can use any validation library you want to validate the fields. For example, you can use zod like this:
Click to see the code
Now that we defined the fields, Wasp knows how to:
Validate the data sent from the client
Save the data to the database
Next, let's see how to customize Auth UI to include those fields.
2. Customizing the Signup Component
Using Custom Signup Component
If you are not using Wasp's Auth UI, you can skip this section. Just make sure to include the extra fields in your custom signup form.
Read more about using the signup actions for:
email auth here
username & password auth here
If you are using Wasp's Auth UI, you can customize the SignupForm component by passing the additionalFields prop to it. It can be either a list of extra fields or a render function.
When you pass in a list of extra fields to the SignupForm, they are added to the form one by one, in the order you pass them in.
Inside the list, there can be either objects or render functions (you can combine them):
Objects are a simple way to describe new fields you need, but a bit less flexible than render functions.
Render functions can be used to render any UI you want, but they require a bit more code. The render functions receive the react-hook-form object and the form state object as arguments.
JavaScript
TypeScript
src/SignupPage.jsx
import {
SignupForm,
FormError,
FormInput,
FormItemGroup,
FormLabel,
} from 'wasp/client/auth'
export const SignupPage = () => {
return (
<SignupForm
additionalFields={[
/* The address field is defined using an object */
{
name: 'address',
label: 'Address',
type: 'input',
validations: {
required: 'Address is required',
},
},
/* The phone number is defined using a render function */
(form, state) => {
return (
<FormItemGroup>
<FormLabel>Phone Number</FormLabel>
<FormInput
{...form.register('phoneNumber', {
required: 'Phone number is required',
})}
disabled={state.isLoading}
/>
{form.formState.errors.phoneNumber && (
<FormError>
{form.formState.errors.phoneNumber.message}
</FormError>
)}
</FormItemGroup>
)
},
]}
/>
)
}
Read more about the extra fields in the API Reference.
Using a Single Render Function
Instead of passing in a list of extra fields, you can pass in a render function which will receive the react-hook-form object and the form state object as arguments. What ever the render function returns, will be rendered below the default fields.
JavaScript
TypeScript
src/SignupPage.jsx
import { SignupForm, FormItemGroup } from 'wasp/client/auth'
export const SignupPage = () => {
return (
<SignupForm
additionalFields={(form, state) => {
const username = form.watch('username')
return (
username && (
<FormItemGroup>
Hello there <strong>{username}</strong> 👋
</FormItemGroup>
)
)
}}
/>
)
}
Read more about the render function in the API Reference.
API Reference
Auth Fields
JavaScript
TypeScript
main.wasp
title: "My app",
//...
auth: {
userEntity: User,
methods: {
usernameAndPassword: {}, // use this or email, not both
email: {}, // use this or usernameAndPassword, not both
google: {},
gitHub: {},
},
onAuthFailedRedirectTo: "/someRoute",
signup: { ... }
}
}
//...
app.auth is a dictionary with the following fields:
userEntity: entity required
The entity representing the user connected to your business logic.
You can read more about how the User entity is connected to the rest of the auth system in the Auth Entities section of the docs.
methods: dict required
A dictionary of auth methods enabled for the app.
Click on each auth method for more details.
onAuthFailedRedirectTo: String required
The route to which Wasp should redirect unauthenticated user when they try to access a private page (i.e., a page that has authRequired: true). Check out these essentials docs on auth to see an example of usage.
onAuthSucceededRedirectTo: String
The route to which Wasp will send a successfully authenticated after a successful login/signup. The default value is "/".
note
Automatic redirect on successful login only works when using the Wasp-provided Auth UI.
signup: SignupOptions
Read more about the signup process customization API in the Signup Fields Customization section.
Signup Fields Customization
If you want to add extra fields to the signup process, the server needs to know how to save them to the database. You do that by defining the auth.methods.{authMethod}.userSignupFields field in your main.wasp file.
JavaScript
TypeScript
main.wasp
app crudTesting {
// ...
auth: {
userEntity: User,
methods: {
usernameAndPassword: {
userSignupFields: import { userSignupFields } from "@src/auth/signup",
},
},
onAuthFailedRedirectTo: "/login",
},
}
Then we'll export the userSignupFields object from the src/auth/signup.js file:
src/auth/signup.js
import { defineUserSignupFields } from 'wasp/server/auth'
export const userSignupFields = defineUserSignupFields({
address: async (data) => {
const address = data.address
if (typeof address !== 'string') {
throw new Error('Address is required')
}
if (address.length < 5) {
throw new Error('Address must be at least 5 characters long')
}
return address
},
})
The userSignupFields object is an object where the keys represent the field name, and the values are functions that receive the data sent from the client* and return the value of the field.
If the value that the function received is invalid, the function should throw an error.
* We exclude the password field from this object to prevent it from being saved as plain text in the database. The password field is handled by Wasp's auth backend.
SignupForm Customization
To customize the SignupForm component, you need to pass in the additionalFields prop. It can be either a list of extra fields or a render function.
JavaScript
TypeScript
src/SignupPage.jsx
import {
SignupForm,
FormError,
FormInput,
FormItemGroup,
FormLabel,
} from 'wasp/client/auth'
export const SignupPage = () => {
return (
<SignupForm
additionalFields={[
{
name: 'address',
label: 'Address',
type: 'input',
validations: {
required: 'Address is required',
},
},
(form, state) => {
return (
<FormItemGroup>
<FormLabel>Phone Number</FormLabel>
<FormInput
{...form.register('phoneNumber', {
required: 'Phone number is required',
})}
disabled={state.isLoading}
/>
{form.formState.errors.phoneNumber && (
<FormError>
{form.formState.errors.phoneNumber.message}
</FormError>
)}
</FormItemGroup>
)
},
]}
/>
)
}
The extra fields can be either objects or render functions (you can combine them):
Objects are a simple way to describe new fields you need, but a bit less flexible than render functions.
The objects have the following properties:
name required
the name of the field
label required
the label of the field (used in the UI)
type required
the type of the field, which can be input or textarea
validations
an object with the validation rules for the field. The keys are the validation names, and the values are the validation error messages. Read more about the available validation rules in the react-hook-form docs.
Render functions receive the react-hook-form object and the form state as arguments, and they can use them to render arbitrary UI elements.
The render function has the following signature:
;(form: UseFormReturn, state: FormState) => React.ReactNode
form required
the react-hook-form object, read more about it in the react-hook-form docs
you need to use the form.register function to register your fields
state required
the form state object which has the following properties:
isLoading: boolean
whether the form is currently submitting | https://wasp-lang.dev/docs/auth/overview |
|
1 | null | 2024-04-23T15:13:55.809Z | https://wasp-lang.dev/docs/auth/email | https://wasp-lang.dev/docs | http | ## Email
Wasp supports e-mail authentication out of the box, along with email verification and "forgot your password?" flows. It provides you with the server-side implementation and email templates for all of these flows.
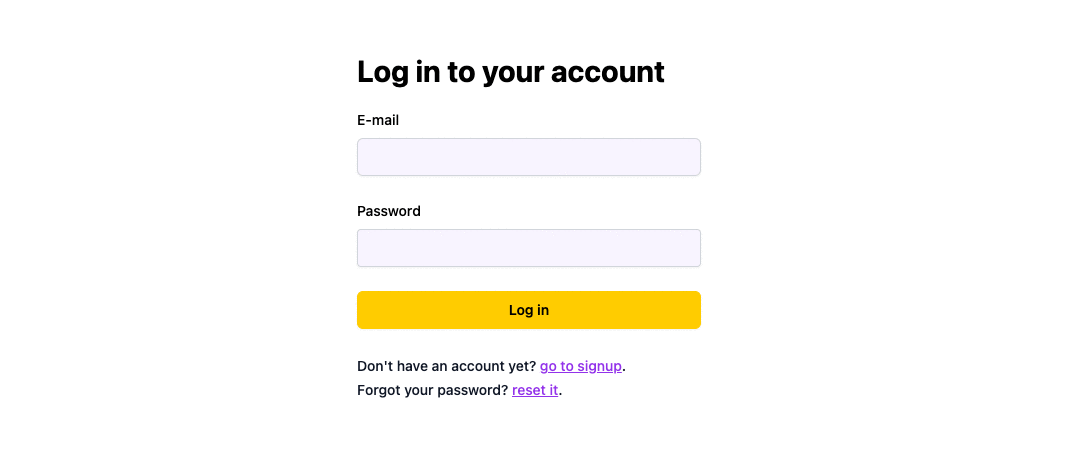
Using multiple auth identities for a single user
Wasp currently doesn't support multiple auth identities for a single user. This means, for example, that a user can't have both an email-based auth identity and a Google-based auth identity. This is something we will add in the future with the introduction of the [account merging feature](https://github.com/wasp-lang/wasp/issues/954).
Account merging means that multiple auth identities can be merged into a single user account. For example, a user's email and Google identity can be merged into a single user account. Then the user can log in with either their email or Google account and they will be logged into the same account.
## Setting Up Email Authentication[](#setting-up-email-authentication "Direct link to Setting Up Email Authentication")
We'll need to take the following steps to set up email authentication:
1. Enable email authentication in the Wasp file
2. Add the `User` entity
3. Add the auth routes and pages
4. Use Auth UI components in our pages
5. Set up the email sender
Structure of the `main.wasp` file we will end up with:
main.wasp
```
// Configuring e-mail authenticationapp myApp { auth: { ... }}// Defining User entityentity User { ... }// Defining routes and pagesroute SignupRoute { ... }page SignupPage { ... }// ...
```
### 1\. Enable Email Authentication in `main.wasp`[](#1-enable-email-authentication-in-mainwasp "Direct link to 1-enable-email-authentication-in-mainwasp")
Let's start with adding the following to our `main.wasp` file:
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { // 1. Specify the user entity (we'll define it next) userEntity: User, methods: { // 2. Enable email authentication email: { // 3. Specify the email from field fromField: { name: "My App Postman", email: "[email protected]" }, // 4. Specify the email verification and password reset options (we'll talk about them later) emailVerification: { clientRoute: EmailVerificationRoute, }, passwordReset: { clientRoute: PasswordResetRoute, }, }, }, onAuthFailedRedirectTo: "/login", onAuthSucceededRedirectTo: "/" },}
```
Read more about the `email` auth method options [here](#fields-in-the-email-dict).
### 2\. Add the User Entity[](#2-add-the-user-entity "Direct link to 2. Add the User Entity")
The `User` entity can be as simple as including only the `id` field:
* JavaScript
* TypeScript
main.wasp
```
// 5. Define the user entityentity User {=psl id Int @id @default(autoincrement()) // Add your own fields below // ...psl=}
```
You can read more about how the `User` entity is connected to the rest of the auth system in the [Auth Entities](https://wasp-lang.dev/docs/auth/entities) section of the docs.
### 3\. Add the Routes and Pages[](#3-add-the-routes-and-pages "Direct link to 3. Add the Routes and Pages")
Next, we need to define the routes and pages for the authentication pages.
Add the following to the `main.wasp` file:
* JavaScript
* TypeScript
main.wasp
```
// ...// 6. Define the routesroute LoginRoute { path: "/login", to: LoginPage }page LoginPage { component: import { Login } from "@src/pages/auth.jsx"}route SignupRoute { path: "/signup", to: SignupPage }page SignupPage { component: import { Signup } from "@src/pages/auth.jsx"}route RequestPasswordResetRoute { path: "/request-password-reset", to: RequestPasswordResetPage }page RequestPasswordResetPage { component: import { RequestPasswordReset } from "@src/pages/auth.jsx",}route PasswordResetRoute { path: "/password-reset", to: PasswordResetPage }page PasswordResetPage { component: import { PasswordReset } from "@src/pages/auth.jsx",}route EmailVerificationRoute { path: "/email-verification", to: EmailVerificationPage }page EmailVerificationPage { component: import { EmailVerification } from "@src/pages/auth.jsx",}
```
We'll define the React components for these pages in the `src/pages/auth.tsx` file below.
### 4\. Create the Client Pages[](#4-create-the-client-pages "Direct link to 4. Create the Client Pages")
info
We are using [Tailwind CSS](https://tailwindcss.com/) to style the pages. Read more about how to add it [here](https://wasp-lang.dev/docs/project/css-frameworks).
Let's create a `auth.tsx` file in the `src/pages` folder and add the following to it:
* JavaScript
* TypeScript
src/pages/auth.jsx
```
import { LoginForm, SignupForm, VerifyEmailForm, ForgotPasswordForm, ResetPasswordForm,} from 'wasp/client/auth'import { Link } from 'react-router-dom'export function Login() { return ( <Layout> <LoginForm /> <br /> <span className="text-sm font-medium text-gray-900"> Don't have an account yet? <Link to="/signup">go to signup</Link>. </span> <br /> <span className="text-sm font-medium text-gray-900"> Forgot your password? <Link to="/request-password-reset">reset it</Link> . </span> </Layout> );}export function Signup() { return ( <Layout> <SignupForm /> <br /> <span className="text-sm font-medium text-gray-900"> I already have an account (<Link to="/login">go to login</Link>). </span> </Layout> );}export function EmailVerification() { return ( <Layout> <VerifyEmailForm /> <br /> <span className="text-sm font-medium text-gray-900"> If everything is okay, <Link to="/login">go to login</Link> </span> </Layout> );}export function RequestPasswordReset() { return ( <Layout> <ForgotPasswordForm /> </Layout> );}export function PasswordReset() { return ( <Layout> <ResetPasswordForm /> <br /> <span className="text-sm font-medium text-gray-900"> If everything is okay, <Link to="/login">go to login</Link> </span> </Layout> );}// A layout component to center the contentexport function Layout({ children }) { return ( <div className="w-full h-full bg-white"> <div className="min-w-full min-h-[75vh] flex items-center justify-center"> <div className="w-full h-full max-w-sm p-5 bg-white"> <div>{children}</div> </div> </div> </div> );}
```
We imported the generated Auth UI components and used them in our pages. Read more about the Auth UI components [here](https://wasp-lang.dev/docs/auth/ui).
### 5\. Set up an Email Sender[](#5-set-up-an-email-sender "Direct link to 5. Set up an Email Sender")
To support e-mail verification and password reset flows, we need an e-mail sender. Luckily, Wasp supports several email providers out of the box.
We'll use the `Dummy` provider to speed up the setup. It just logs the emails to the console instead of sending them. You can use any of the [supported email providers](https://wasp-lang.dev/docs/advanced/email#providers).
To set up the `Dummy` provider to send emails, add the following to the `main.wasp` file:
* JavaScript
* TypeScript
main.wasp
```
app myApp { // ... // 7. Set up the email sender emailSender: { provider: Dummy, }}
```
### Conclusion[](#conclusion "Direct link to Conclusion")
That's it! We have set up email authentication in our app. 🎉
Running `wasp db migrate-dev` and then `wasp start` should give you a working app with email authentication. If you want to put some of the pages behind authentication, read the [auth overview](https://wasp-lang.dev/docs/auth/overview).
## Login and Signup Flows[](#login-and-signup-flows "Direct link to Login and Signup Flows")
### Login[](#login "Direct link to Login")
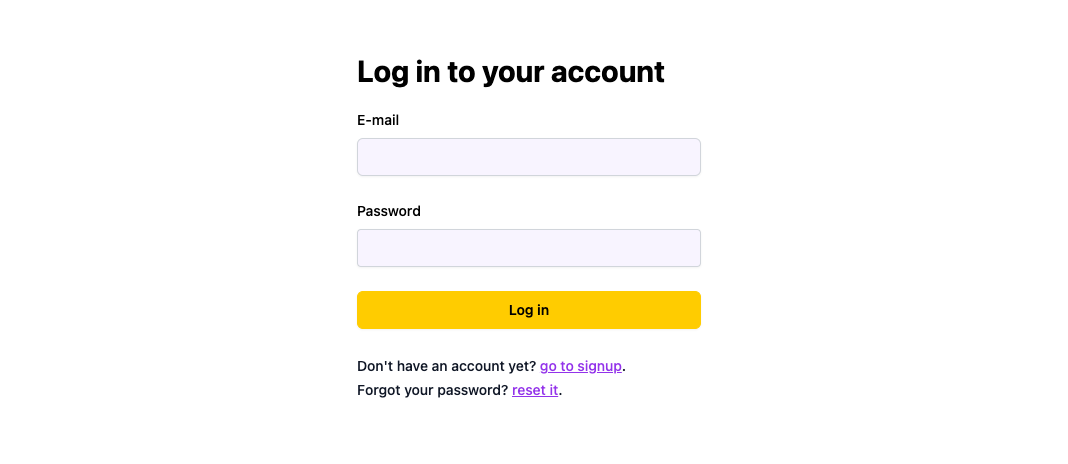
### Signup[](#signup "Direct link to Signup")
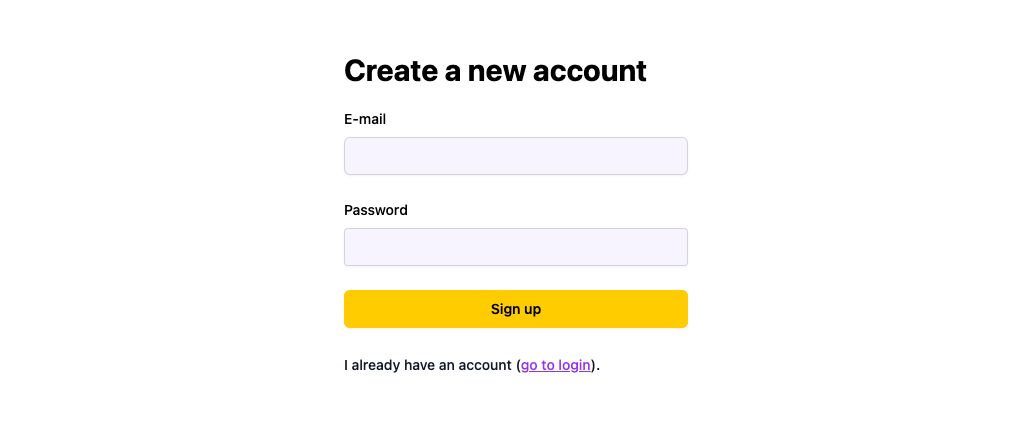
Some of the behavior you get out of the box:
1. Rate limiting
We are limiting the rate of sign-up requests to **1 request per minute** per email address. This is done to prevent spamming.
2. Preventing user email leaks
If somebody tries to signup with an email that already exists and it's verified, we _pretend_ that the account was created instead of saying it's an existing account. This is done to prevent leaking the user's email address.
3. Allowing registration for unverified emails
If a user tries to register with an existing but **unverified** email, we'll allow them to do that. This is done to prevent bad actors from locking out other users from registering with their email address.
4. Password validation
Read more about the default password validation rules and how to override them in [auth overview docs](https://wasp-lang.dev/docs/auth/overview).
## Email Verification Flow[](#email-verification-flow "Direct link to Email Verification Flow")
Automatic email verification in development
In development mode, you can skip the email verification step by setting the `SKIP_EMAIL_VERIFICATION_IN_DEV` environment variable to `true` in your `.env.server` file:
.env.server
```
SKIP_EMAIL_VERIFICATION_IN_DEV=true
```
This is useful when you are developing your app and don't want to go through the email verification flow every time you sign up. It can be also useful when you are writing automated tests for your app.
By default, Wasp requires the e-mail to be verified before allowing the user to log in. This is done by sending a verification email to the user's email address and requiring the user to click on a link in the email to verify their email address.
Our setup looks like this:
* JavaScript
* TypeScript
main.wasp
```
// ...emailVerification: { clientRoute: EmailVerificationRoute,}
```
When the user receives an e-mail, they receive a link that goes to the client route specified in the `clientRoute` field. In our case, this is the `EmailVerificationRoute` route we defined in the `main.wasp` file.
The content of the e-mail can be customized, read more about it [here](#emailverification-emailverificationconfig-).
### Email Verification Page[](#email-verification-page "Direct link to Email Verification Page")
We defined our email verification page in the `auth.tsx` file.
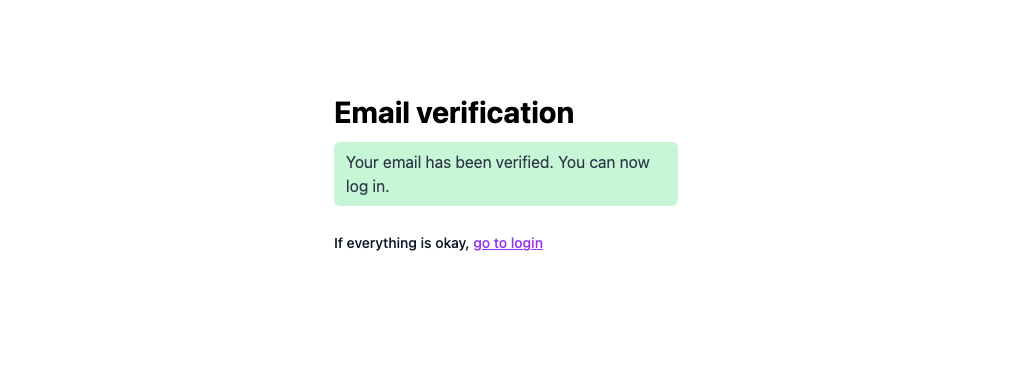
## Password Reset Flow[](#password-reset-flow "Direct link to Password Reset Flow")
Users can request a password and then they'll receive an e-mail with a link to reset their password.
Some of the behavior you get out of the box:
1. Rate limiting
We are limiting the rate of sign-up requests to **1 request per minute** per email address. This is done to prevent spamming.
2. Preventing user email leaks
If somebody requests a password reset with an unknown email address, we'll give back the same response as if the user requested a password reset successfully. This is done to prevent leaking information.
Our setup in `main.wasp` looks like this:
* JavaScript
* TypeScript
main.wasp
```
// ...passwordReset: { clientRoute: PasswordResetRoute,}
```
### Request Password Reset Page[](#request-password-reset-page "Direct link to Request Password Reset Page")
Users request their password to be reset by going to the `/request-password-reset` route. We defined our request password reset page in the `auth.tsx` file.
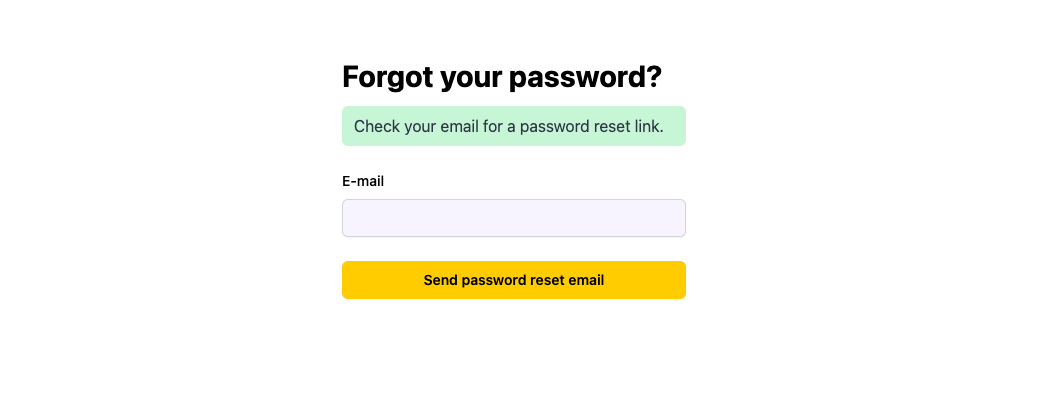
### Password Reset Page[](#password-reset-page "Direct link to Password Reset Page")
When the user receives an e-mail, they receive a link that goes to the client route specified in the `clientRoute` field. In our case, this is the `PasswordResetRoute` route we defined in the `main.wasp` file.
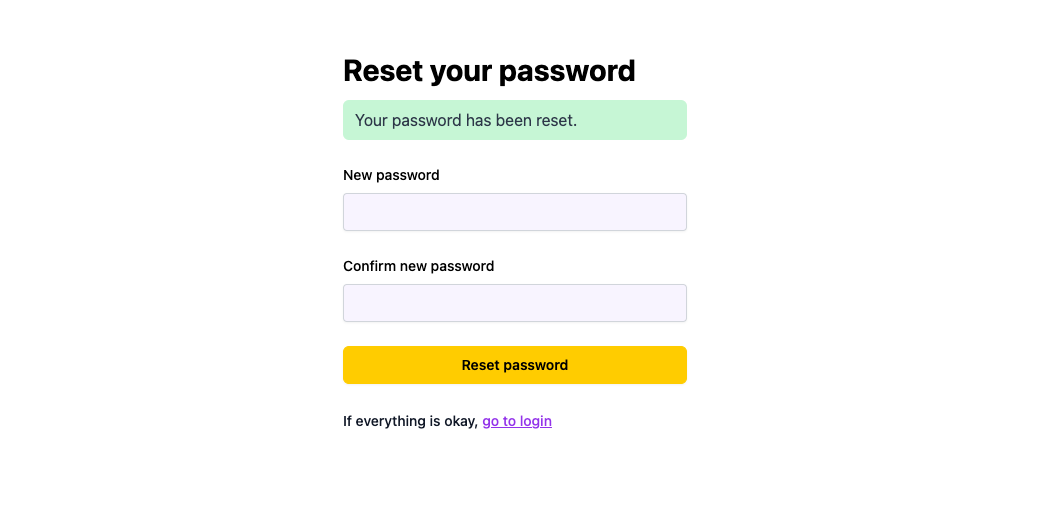
Users can enter their new password there.
The content of the e-mail can be customized, read more about it [here](#passwordreset-passwordresetconfig-).
## Creating a Custom Sign-up Action[](#creating-a-custom-sign-up-action "Direct link to Creating a Custom Sign-up Action")
Creating a custom sign-up action
We don't recommend creating a custom sign-up action unless you have a good reason to do so. It is a complex process and you can easily make a mistake that will compromise the security of your app.
The code of your custom sign-up action can look like this:
* JavaScript
* TypeScript
main.wasp
```
// ...action customSignup { fn: import { signup } from "@src/auth/signup.js",}
```
src/auth/signup.js
```
import { ensurePasswordIsPresent, ensureValidPassword, ensureValidEmail, createProviderId, sanitizeAndSerializeProviderData, deserializeAndSanitizeProviderData, findAuthIdentity, createUser, createEmailVerificationLink, sendEmailVerificationEmail,} from 'wasp/server/auth'export const signup = async (args, _context) => { ensureValidEmail(args) ensurePasswordIsPresent(args) ensureValidPassword(args) try { const providerId = createProviderId('email', args.email) const existingAuthIdentity = await findAuthIdentity(providerId) if (existingAuthIdentity) { const providerData = deserializeAndSanitizeProviderData(existingAuthIdentity.providerData) // Your custom code here } else { // sanitizeAndSerializeProviderData will hash the user's password const newUserProviderData = await sanitizeAndSerializeProviderData({ hashedPassword: args.password, isEmailVerified: false, emailVerificationSentAt: null, passwordResetSentAt: null, }) await createUser( providerId, providerData, // Any additional data you want to store on the User entity {}, ) // Verification link links to a client route e.g. /email-verification const verificationLink = await createEmailVerificationLink(args.email, '/email-verification'); try { await sendEmailVerificationEmail( args.email, { from: { name: "My App Postman", email: "[email protected]", }, to: args.email, subject: "Verify your email", text: `Click the link below to verify your email: ${verificationLink}`, html: ` <p>Click the link below to verify your email</p> <a href="${verificationLink}">Verify email</a> `, } ); } catch (e: unknown) { console.error("Failed to send email verification email:", e); throw new HttpError(500, "Failed to send email verification email."); } } } catch (e) { return { success: false, message: e.message, } } // Your custom code after sign-up. // ... return { success: true, message: 'User created successfully', }}
```
We suggest using the built-in field validators for your authentication flow. You can import them from `wasp/server/auth`. These are the same validators that Wasp uses internally for the default authentication flow.
#### Email[](#email "Direct link to Email")
* `ensureValidEmail(args)`
Checks if the email is valid and throws an error if it's not. Read more about the validation rules [here](https://wasp-lang.dev/docs/auth/overview#default-validations).
#### Password[](#password "Direct link to Password")
* `ensurePasswordIsPresent(args)`
Checks if the password is present and throws an error if it's not.
* `ensureValidPassword(args)`
Checks if the password is valid and throws an error if it's not. Read more about the validation rules [here](https://wasp-lang.dev/docs/auth/overview#default-validations).
## Using Auth[](#using-auth "Direct link to Using Auth")
To read more about how to set up the logout button and how to get access to the logged-in user in our client and server code, read the [auth overview docs](https://wasp-lang.dev/docs/auth/overview).
### `getEmail`[](#getemail "Direct link to getemail")
If you are looking to access the user's email in your code, you can do that by accessing the info about the user that is stored in the `user.auth.identities` array.
To make things a bit easier for you, Wasp offers the `getEmail` helper.
The `getEmail` helper returns the user's email or `null` if the user doesn't have an email auth identity.
* JavaScript
* TypeScript
src/MainPage.jsx
```
import { getEmail } from 'wasp/auth'const MainPage = ({ user }) => { const email = getEmail(user) // ...}
```
src/tasks.js
```
import { getEmail } from 'wasp/auth'export const createTask = async (args, context) => { const email = getEmail(context.user) // ...}
```
## API Reference[](#api-reference "Direct link to API Reference")
Let's go over the options we can specify when using email authentication.
### `userEntity` fields[](#userentity-fields "Direct link to userentity-fields")
* JavaScript
* TypeScript
main.wasp
```
app myApp { title: "My app", // ... auth: { userEntity: User, methods: { email: { // We'll explain these options below }, }, onAuthFailedRedirectTo: "/someRoute" }, // ...}entity User {=psl id Int @id @default(autoincrement())psl=}
```
The user entity needs to have the following fields:
* `id` required
It can be of any type, but it needs to be marked with `@id`
You can add any other fields you want to the user entity. Make sure to also define them in the `userSignupFields` field if they need to be set during the sign-up process.
### Fields in the `email` dict[](#fields-in-the-email-dict "Direct link to fields-in-the-email-dict")
* JavaScript
* TypeScript
main.wasp
```
app myApp { title: "My app", // ... auth: { userEntity: User, methods: { email: { userSignupFields: import { userSignupFields } from "@src/auth.js", fromField: { name: "My App", email: "[email protected]" }, emailVerification: { clientRoute: EmailVerificationRoute, getEmailContentFn: import { getVerificationEmailContent } from "@src/auth/email.js", }, passwordReset: { clientRoute: PasswordResetRoute, getEmailContentFn: import { getPasswordResetEmailContent } from "@src/auth/email.js", }, }, }, onAuthFailedRedirectTo: "/someRoute" }, // ...}
```
#### `userSignupFields: ExtImport`[](#usersignupfields-extimport "Direct link to usersignupfields-extimport")
`userSignupFields` defines all the extra fields that need to be set on the `User` during the sign-up process. For example, if you have `address` and `phone` fields on your `User` entity, you can set them by defining the `userSignupFields` like this:
* JavaScript
* TypeScript
src/auth.js
```
import { defineUserSignupFields } from 'wasp/server/auth'export const userSignupFields = defineUserSignupFields({ address: (data) => { if (!data.address) { throw new Error('Address is required') } return data.address } phone: (data) => data.phone,})
```
Read more about the \`userSignupFields\` function \[here\](./overview#1-defining-extra-fields).
#### `fromField: EmailFromField` required[](#fromfield-emailfromfield- "Direct link to fromfield-emailfromfield-")
`fromField` is a dict that specifies the name and e-mail address of the sender of the e-mails sent by your app.
It has the following fields:
* `name`: name of the sender
* `email`: e-mail address of the sender required
#### `emailVerification: EmailVerificationConfig` required[](#emailverification-emailverificationconfig- "Direct link to emailverification-emailverificationconfig-")
`emailVerification` is a dict that specifies the details of the e-mail verification process.
It has the following fields:
* `clientRoute: Route`: a route that is used for the user to verify their e-mail address. required
Client route should handle the process of taking a token from the URL and sending it to the server to verify the e-mail address. You can use our `verifyEmail` action for that.
* JavaScript
* TypeScript
src/pages/EmailVerificationPage.jsx
```
import { verifyEmail } from 'wasp/client/auth'...await verifyEmail({ token });
```
note
We used Auth UI above to avoid doing this work of sending the token to the server manually.
* `getEmailContentFn: ExtImport`: a function that returns the content of the e-mail that is sent to the user.
Defining `getEmailContentFn` can be done by defining a file in the `src` directory.
* JavaScript
* TypeScript
src/email.js
```
export const getVerificationEmailContent = ({ verificationLink }) => ({ subject: 'Verify your email', text: `Click the link below to verify your email: ${verificationLink}`, html: ` <p>Click the link below to verify your email</p> <a href="${verificationLink}">Verify email</a> `,})
```
This is the default content of the e-mail, you can customize it to your liking.
#### `passwordReset: PasswordResetConfig` required[](#passwordreset-passwordresetconfig- "Direct link to passwordreset-passwordresetconfig-")
`passwordReset` is a dict that specifies the password reset process.
It has the following fields:
* `clientRoute: Route`: a route that is used for the user to reset their password. required
Client route should handle the process of taking a token from the URL and a new password from the user and sending it to the server. You can use our `requestPasswordReset` and `resetPassword` actions to do that.
* JavaScript
* TypeScript
src/pages/ForgotPasswordPage.jsx
```
import { requestPasswordReset } from 'wasp/client/auth'...await requestPasswordReset({ email });
```
src/pages/PasswordResetPage.jsx
```
import { resetPassword } from 'wasp/client/auth'...await resetPassword({ password, token })
```
note
We used Auth UI above to avoid doing this work of sending the password request and the new password to the server manually.
* `getEmailContentFn: ExtImport`: a function that returns the content of the e-mail that is sent to the user.
Defining `getEmailContentFn` is done by defining a function that looks like this:
* JavaScript
* TypeScript
src/email.js
```
export const getPasswordResetEmailContent = ({ passwordResetLink }) => ({ subject: 'Password reset', text: `Click the link below to reset your password: ${passwordResetLink}`, html: ` <p>Click the link below to reset your password</p> <a href="${passwordResetLink}">Reset password</a> `,})
```
This is the default content of the e-mail, you can customize it to your liking. | null | https://wasp-lang.dev/docs/auth/email | Wasp supports e-mail authentication out of the box, along with email verification and "forgot your password?" flows. It provides you with the server-side implementation and email templates for all of these flows. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecce24e983982-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:13:55 GMT | Tue, 23 Apr 2024 15:23:55 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=VIQeKF6tWOVy24y5pOdxcPQgWhS4SLYgbmzwh1pEEaPlxkdI%2BSLgCSwd%2BdoxhthaBPo6FLgsBHVcMB9bEmF2J4KFbL3wTBTgK4FcLn2NFqsoVYOP%2FGSFAOvMDFxSAP7B"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 1ae5506f73c980f040e9601147119c39d061ad23 | null | 44E4:651DA:3D35A3C:485664D:6627D032 | HIT | MISS | cache-iad-kiad7000099-IAD | S1713885236.592105,VS0,VE23 | null | null | en | og:image | https://wasp-lang.dev/docs/auth/email | og:url | Email | Wasp | og:title | Wasp supports e-mail authentication out of the box, along with email verification and "forgot your password?" flows. It provides you with the server-side implementation and email templates for all of these flows. | og:description | Email | Wasp | null | Email
Wasp supports e-mail authentication out of the box, along with email verification and "forgot your password?" flows. It provides you with the server-side implementation and email templates for all of these flows.
Using multiple auth identities for a single user
Wasp currently doesn't support multiple auth identities for a single user. This means, for example, that a user can't have both an email-based auth identity and a Google-based auth identity. This is something we will add in the future with the introduction of the account merging feature.
Account merging means that multiple auth identities can be merged into a single user account. For example, a user's email and Google identity can be merged into a single user account. Then the user can log in with either their email or Google account and they will be logged into the same account.
Setting Up Email Authentication
We'll need to take the following steps to set up email authentication:
Enable email authentication in the Wasp file
Add the User entity
Add the auth routes and pages
Use Auth UI components in our pages
Set up the email sender
Structure of the main.wasp file we will end up with:
main.wasp
// Configuring e-mail authentication
app myApp {
auth: { ... }
}
// Defining User entity
entity User { ... }
// Defining routes and pages
route SignupRoute { ... }
page SignupPage { ... }
// ...
1. Enable Email Authentication in main.wasp
Let's start with adding the following to our main.wasp file:
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
// 1. Specify the user entity (we'll define it next)
userEntity: User,
methods: {
// 2. Enable email authentication
email: {
// 3. Specify the email from field
fromField: {
name: "My App Postman",
email: "[email protected]"
},
// 4. Specify the email verification and password reset options (we'll talk about them later)
emailVerification: {
clientRoute: EmailVerificationRoute,
},
passwordReset: {
clientRoute: PasswordResetRoute,
},
},
},
onAuthFailedRedirectTo: "/login",
onAuthSucceededRedirectTo: "/"
},
}
Read more about the email auth method options here.
2. Add the User Entity
The User entity can be as simple as including only the id field:
JavaScript
TypeScript
main.wasp
// 5. Define the user entity
entity User {=psl
id Int @id @default(autoincrement())
// Add your own fields below
// ...
psl=}
You can read more about how the User entity is connected to the rest of the auth system in the Auth Entities section of the docs.
3. Add the Routes and Pages
Next, we need to define the routes and pages for the authentication pages.
Add the following to the main.wasp file:
JavaScript
TypeScript
main.wasp
// ...
// 6. Define the routes
route LoginRoute { path: "/login", to: LoginPage }
page LoginPage {
component: import { Login } from "@src/pages/auth.jsx"
}
route SignupRoute { path: "/signup", to: SignupPage }
page SignupPage {
component: import { Signup } from "@src/pages/auth.jsx"
}
route RequestPasswordResetRoute { path: "/request-password-reset", to: RequestPasswordResetPage }
page RequestPasswordResetPage {
component: import { RequestPasswordReset } from "@src/pages/auth.jsx",
}
route PasswordResetRoute { path: "/password-reset", to: PasswordResetPage }
page PasswordResetPage {
component: import { PasswordReset } from "@src/pages/auth.jsx",
}
route EmailVerificationRoute { path: "/email-verification", to: EmailVerificationPage }
page EmailVerificationPage {
component: import { EmailVerification } from "@src/pages/auth.jsx",
}
We'll define the React components for these pages in the src/pages/auth.tsx file below.
4. Create the Client Pages
info
We are using Tailwind CSS to style the pages. Read more about how to add it here.
Let's create a auth.tsx file in the src/pages folder and add the following to it:
JavaScript
TypeScript
src/pages/auth.jsx
import {
LoginForm,
SignupForm,
VerifyEmailForm,
ForgotPasswordForm,
ResetPasswordForm,
} from 'wasp/client/auth'
import { Link } from 'react-router-dom'
export function Login() {
return (
<Layout>
<LoginForm />
<br />
<span className="text-sm font-medium text-gray-900">
Don't have an account yet? <Link to="/signup">go to signup</Link>.
</span>
<br />
<span className="text-sm font-medium text-gray-900">
Forgot your password? <Link to="/request-password-reset">reset it</Link>
.
</span>
</Layout>
);
}
export function Signup() {
return (
<Layout>
<SignupForm />
<br />
<span className="text-sm font-medium text-gray-900">
I already have an account (<Link to="/login">go to login</Link>).
</span>
</Layout>
);
}
export function EmailVerification() {
return (
<Layout>
<VerifyEmailForm />
<br />
<span className="text-sm font-medium text-gray-900">
If everything is okay, <Link to="/login">go to login</Link>
</span>
</Layout>
);
}
export function RequestPasswordReset() {
return (
<Layout>
<ForgotPasswordForm />
</Layout>
);
}
export function PasswordReset() {
return (
<Layout>
<ResetPasswordForm />
<br />
<span className="text-sm font-medium text-gray-900">
If everything is okay, <Link to="/login">go to login</Link>
</span>
</Layout>
);
}
// A layout component to center the content
export function Layout({ children }) {
return (
<div className="w-full h-full bg-white">
<div className="min-w-full min-h-[75vh] flex items-center justify-center">
<div className="w-full h-full max-w-sm p-5 bg-white">
<div>{children}</div>
</div>
</div>
</div>
);
}
We imported the generated Auth UI components and used them in our pages. Read more about the Auth UI components here.
5. Set up an Email Sender
To support e-mail verification and password reset flows, we need an e-mail sender. Luckily, Wasp supports several email providers out of the box.
We'll use the Dummy provider to speed up the setup. It just logs the emails to the console instead of sending them. You can use any of the supported email providers.
To set up the Dummy provider to send emails, add the following to the main.wasp file:
JavaScript
TypeScript
main.wasp
app myApp {
// ...
// 7. Set up the email sender
emailSender: {
provider: Dummy,
}
}
Conclusion
That's it! We have set up email authentication in our app. 🎉
Running wasp db migrate-dev and then wasp start should give you a working app with email authentication. If you want to put some of the pages behind authentication, read the auth overview.
Login and Signup Flows
Login
Signup
Some of the behavior you get out of the box:
Rate limiting
We are limiting the rate of sign-up requests to 1 request per minute per email address. This is done to prevent spamming.
Preventing user email leaks
If somebody tries to signup with an email that already exists and it's verified, we pretend that the account was created instead of saying it's an existing account. This is done to prevent leaking the user's email address.
Allowing registration for unverified emails
If a user tries to register with an existing but unverified email, we'll allow them to do that. This is done to prevent bad actors from locking out other users from registering with their email address.
Password validation
Read more about the default password validation rules and how to override them in auth overview docs.
Email Verification Flow
Automatic email verification in development
In development mode, you can skip the email verification step by setting the SKIP_EMAIL_VERIFICATION_IN_DEV environment variable to true in your .env.server file:
.env.server
SKIP_EMAIL_VERIFICATION_IN_DEV=true
This is useful when you are developing your app and don't want to go through the email verification flow every time you sign up. It can be also useful when you are writing automated tests for your app.
By default, Wasp requires the e-mail to be verified before allowing the user to log in. This is done by sending a verification email to the user's email address and requiring the user to click on a link in the email to verify their email address.
Our setup looks like this:
JavaScript
TypeScript
main.wasp
// ...
emailVerification: {
clientRoute: EmailVerificationRoute,
}
When the user receives an e-mail, they receive a link that goes to the client route specified in the clientRoute field. In our case, this is the EmailVerificationRoute route we defined in the main.wasp file.
The content of the e-mail can be customized, read more about it here.
Email Verification Page
We defined our email verification page in the auth.tsx file.
Password Reset Flow
Users can request a password and then they'll receive an e-mail with a link to reset their password.
Some of the behavior you get out of the box:
Rate limiting
We are limiting the rate of sign-up requests to 1 request per minute per email address. This is done to prevent spamming.
Preventing user email leaks
If somebody requests a password reset with an unknown email address, we'll give back the same response as if the user requested a password reset successfully. This is done to prevent leaking information.
Our setup in main.wasp looks like this:
JavaScript
TypeScript
main.wasp
// ...
passwordReset: {
clientRoute: PasswordResetRoute,
}
Request Password Reset Page
Users request their password to be reset by going to the /request-password-reset route. We defined our request password reset page in the auth.tsx file.
Password Reset Page
When the user receives an e-mail, they receive a link that goes to the client route specified in the clientRoute field. In our case, this is the PasswordResetRoute route we defined in the main.wasp file.
Users can enter their new password there.
The content of the e-mail can be customized, read more about it here.
Creating a Custom Sign-up Action
Creating a custom sign-up action
We don't recommend creating a custom sign-up action unless you have a good reason to do so. It is a complex process and you can easily make a mistake that will compromise the security of your app.
The code of your custom sign-up action can look like this:
JavaScript
TypeScript
main.wasp
// ...
action customSignup {
fn: import { signup } from "@src/auth/signup.js",
}
src/auth/signup.js
import {
ensurePasswordIsPresent,
ensureValidPassword,
ensureValidEmail,
createProviderId,
sanitizeAndSerializeProviderData,
deserializeAndSanitizeProviderData,
findAuthIdentity,
createUser,
createEmailVerificationLink,
sendEmailVerificationEmail,
} from 'wasp/server/auth'
export const signup = async (args, _context) => {
ensureValidEmail(args)
ensurePasswordIsPresent(args)
ensureValidPassword(args)
try {
const providerId = createProviderId('email', args.email)
const existingAuthIdentity = await findAuthIdentity(providerId)
if (existingAuthIdentity) {
const providerData = deserializeAndSanitizeProviderData(existingAuthIdentity.providerData)
// Your custom code here
} else {
// sanitizeAndSerializeProviderData will hash the user's password
const newUserProviderData = await sanitizeAndSerializeProviderData({
hashedPassword: args.password,
isEmailVerified: false,
emailVerificationSentAt: null,
passwordResetSentAt: null,
})
await createUser(
providerId,
providerData,
// Any additional data you want to store on the User entity
{},
)
// Verification link links to a client route e.g. /email-verification
const verificationLink = await createEmailVerificationLink(args.email, '/email-verification');
try {
await sendEmailVerificationEmail(
args.email,
{
from: {
name: "My App Postman",
email: "[email protected]",
},
to: args.email,
subject: "Verify your email",
text: `Click the link below to verify your email: ${verificationLink}`,
html: `
<p>Click the link below to verify your email</p>
<a href="${verificationLink}">Verify email</a>
`,
}
);
} catch (e: unknown) {
console.error("Failed to send email verification email:", e);
throw new HttpError(500, "Failed to send email verification email.");
}
}
} catch (e) {
return {
success: false,
message: e.message,
}
}
// Your custom code after sign-up.
// ...
return {
success: true,
message: 'User created successfully',
}
}
We suggest using the built-in field validators for your authentication flow. You can import them from wasp/server/auth. These are the same validators that Wasp uses internally for the default authentication flow.
Email
ensureValidEmail(args)
Checks if the email is valid and throws an error if it's not. Read more about the validation rules here.
Password
ensurePasswordIsPresent(args)
Checks if the password is present and throws an error if it's not.
ensureValidPassword(args)
Checks if the password is valid and throws an error if it's not. Read more about the validation rules here.
Using Auth
To read more about how to set up the logout button and how to get access to the logged-in user in our client and server code, read the auth overview docs.
getEmail
If you are looking to access the user's email in your code, you can do that by accessing the info about the user that is stored in the user.auth.identities array.
To make things a bit easier for you, Wasp offers the getEmail helper.
The getEmail helper returns the user's email or null if the user doesn't have an email auth identity.
JavaScript
TypeScript
src/MainPage.jsx
import { getEmail } from 'wasp/auth'
const MainPage = ({ user }) => {
const email = getEmail(user)
// ...
}
src/tasks.js
import { getEmail } from 'wasp/auth'
export const createTask = async (args, context) => {
const email = getEmail(context.user)
// ...
}
API Reference
Let's go over the options we can specify when using email authentication.
userEntity fields
JavaScript
TypeScript
main.wasp
app myApp {
title: "My app",
// ...
auth: {
userEntity: User,
methods: {
email: {
// We'll explain these options below
},
},
onAuthFailedRedirectTo: "/someRoute"
},
// ...
}
entity User {=psl
id Int @id @default(autoincrement())
psl=}
The user entity needs to have the following fields:
id required
It can be of any type, but it needs to be marked with @id
You can add any other fields you want to the user entity. Make sure to also define them in the userSignupFields field if they need to be set during the sign-up process.
Fields in the email dict
JavaScript
TypeScript
main.wasp
app myApp {
title: "My app",
// ...
auth: {
userEntity: User,
methods: {
email: {
userSignupFields: import { userSignupFields } from "@src/auth.js",
fromField: {
name: "My App",
email: "[email protected]"
},
emailVerification: {
clientRoute: EmailVerificationRoute,
getEmailContentFn: import { getVerificationEmailContent } from "@src/auth/email.js",
},
passwordReset: {
clientRoute: PasswordResetRoute,
getEmailContentFn: import { getPasswordResetEmailContent } from "@src/auth/email.js",
},
},
},
onAuthFailedRedirectTo: "/someRoute"
},
// ...
}
userSignupFields: ExtImport
userSignupFields defines all the extra fields that need to be set on the User during the sign-up process. For example, if you have address and phone fields on your User entity, you can set them by defining the userSignupFields like this:
JavaScript
TypeScript
src/auth.js
import { defineUserSignupFields } from 'wasp/server/auth'
export const userSignupFields = defineUserSignupFields({
address: (data) => {
if (!data.address) {
throw new Error('Address is required')
}
return data.address
}
phone: (data) => data.phone,
})
Read more about the `userSignupFields` function [here](./overview#1-defining-extra-fields).
fromField: EmailFromField required
fromField is a dict that specifies the name and e-mail address of the sender of the e-mails sent by your app.
It has the following fields:
name: name of the sender
email: e-mail address of the sender required
emailVerification: EmailVerificationConfig required
emailVerification is a dict that specifies the details of the e-mail verification process.
It has the following fields:
clientRoute: Route: a route that is used for the user to verify their e-mail address. required
Client route should handle the process of taking a token from the URL and sending it to the server to verify the e-mail address. You can use our verifyEmail action for that.
JavaScript
TypeScript
src/pages/EmailVerificationPage.jsx
import { verifyEmail } from 'wasp/client/auth'
...
await verifyEmail({ token });
note
We used Auth UI above to avoid doing this work of sending the token to the server manually.
getEmailContentFn: ExtImport: a function that returns the content of the e-mail that is sent to the user.
Defining getEmailContentFn can be done by defining a file in the src directory.
JavaScript
TypeScript
src/email.js
export const getVerificationEmailContent = ({ verificationLink }) => ({
subject: 'Verify your email',
text: `Click the link below to verify your email: ${verificationLink}`,
html: `
<p>Click the link below to verify your email</p>
<a href="${verificationLink}">Verify email</a>
`,
})
This is the default content of the e-mail, you can customize it to your liking.
passwordReset: PasswordResetConfig required
passwordReset is a dict that specifies the password reset process.
It has the following fields:
clientRoute: Route: a route that is used for the user to reset their password. required
Client route should handle the process of taking a token from the URL and a new password from the user and sending it to the server. You can use our requestPasswordReset and resetPassword actions to do that.
JavaScript
TypeScript
src/pages/ForgotPasswordPage.jsx
import { requestPasswordReset } from 'wasp/client/auth'
...
await requestPasswordReset({ email });
src/pages/PasswordResetPage.jsx
import { resetPassword } from 'wasp/client/auth'
...
await resetPassword({ password, token })
note
We used Auth UI above to avoid doing this work of sending the password request and the new password to the server manually.
getEmailContentFn: ExtImport: a function that returns the content of the e-mail that is sent to the user.
Defining getEmailContentFn is done by defining a function that looks like this:
JavaScript
TypeScript
src/email.js
export const getPasswordResetEmailContent = ({ passwordResetLink }) => ({
subject: 'Password reset',
text: `Click the link below to reset your password: ${passwordResetLink}`,
html: `
<p>Click the link below to reset your password</p>
<a href="${passwordResetLink}">Reset password</a>
`,
})
This is the default content of the e-mail, you can customize it to your liking. | https://wasp-lang.dev/docs/auth/email |
|
1 | null | 2024-04-23T15:14:04.506Z | https://wasp-lang.dev/docs/auth/social-auth/overview | https://wasp-lang.dev/docs | http | ## Overview
Social login options (e.g., _Log in with Google_) are a great (maybe even the best) solution for handling user accounts. A famous old developer joke tells us _"The best auth system is the one you never have to make."_
Wasp wants to make adding social login options to your app as painless as possible.
Using different social providers gives users a chance to sign into your app via their existing accounts on other platforms (Google, GitHub, etc.).
This page goes through the common behaviors between all supported social login providers and shows you how to customize them. It also gives an overview of Wasp's UI helpers - the quickest possible way to get started with social auth.
## Available Providers[](#available-providers "Direct link to Available Providers")
Wasp currently supports the following social login providers:
## User Entity[](#user-entity "Direct link to User Entity")
Wasp requires you to declare a `userEntity` for all `auth` methods (social or otherwise). This field tells Wasp which Entity represents the user.
Here's what the full setup looks like:
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { google: {} }, onAuthFailedRedirectTo: "/login" },}entity User {=psl id Int @id @default(autoincrement()) //...psl=}
```
To learn more about what the fields on these entities represent, look at the [API Reference](#api-reference).
## Default Behavior[](#default-behavior "Direct link to Default Behavior")
When a user **signs in for the first time**, Wasp creates a new user account and links it to the chosen auth provider account for future logins.
## Overrides[](#overrides "Direct link to Overrides")
By default, Wasp doesn't store any information it receives from the social login provider. It only stores the user's ID specific to the provider.
If you wish to store more information about the user, you can override the default behavior. You can do this by defining the `userSignupFields` and `configFn` fields in `main.wasp` for each provider.
You can create custom signup setups, such as allowing users to define a custom username after they sign up with a social provider.
### Example: Allowing User to Set Their Username[](#example-allowing-user-to-set-their-username "Direct link to Example: Allowing User to Set Their Username")
If you want to modify the signup flow (e.g., let users choose their own usernames), you will need to go through three steps:
1. The first step is adding a `isSignupComplete` property to your `User` Entity. This field will signal whether the user has completed the signup process.
2. The second step is overriding the default signup behavior.
3. The third step is implementing the rest of your signup flow and redirecting users where appropriate.
Let's go through both steps in more detail.
#### 1\. Adding the `isSignupComplete` Field to the `User` Entity[](#1-adding-the-issignupcomplete-field-to-the-user-entity "Direct link to 1-adding-the-issignupcomplete-field-to-the-user-entity")
* JavaScript
* TypeScript
main.wasp
```
entity User {=psl id Int @id @default(autoincrement()) username String? @unique isSignupComplete Boolean @default(false)psl=}
```
#### 2\. Overriding the Default Behavior[](#2-overriding-the-default-behavior "Direct link to 2. Overriding the Default Behavior")
Declare an import under `app.auth.methods.google.userSignupFields` (the example assumes you're using Google):
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { google: { userSignupFields: import { userSignupFields } from "@src/auth/google.js" } }, onAuthFailedRedirectTo: "/login" },}// ...
```
And implement the imported function.
src/auth/google.js
```
export const userSignupFields = { isSignupComplete: () => false,}
```
#### 3\. Showing the Correct State on the Client[](#3-showing-the-correct-state-on-the-client "Direct link to 3. Showing the Correct State on the Client")
You can query the user's `isSignupComplete` flag on the client with the [`useAuth()`](https://wasp-lang.dev/docs/auth/overview) hook. Depending on the flag's value, you can redirect users to the appropriate signup step.
For example:
1. When the user lands on the homepage, check the value of `user.isSignupComplete`.
2. If it's `false`, it means the user has started the signup process but hasn't yet chosen their username. Therefore, you can redirect them to `EditUserDetailsPage` where they can edit the `username` property.
* JavaScript
* TypeScript
src/HomePage.jsx
```
import { useAuth } from 'wasp/client/auth'import { Redirect } from 'react-router-dom'export function HomePage() { const { data: user } = useAuth() if (user.isSignupComplete === false) { return <Redirect to="/edit-user-details" /> } // ...}
```
### Using the User's Provider Account Details[](#using-the-users-provider-account-details "Direct link to Using the User's Provider Account Details")
Account details are provider-specific. Each provider has their own rules for defining the `userSignupFields` and `configFn` fields:
## UI Helpers[](#ui-helpers "Direct link to UI Helpers")
Use Auth UI
[Auth UI](https://wasp-lang.dev/docs/auth/ui) is a common name for all high-level auth forms that come with Wasp.
These include fully functional auto-generated login and signup forms with working social login buttons. If you're looking for the fastest way to get your auth up and running, that's where you should look.
The UI helpers described below are lower-level and are useful for creating your custom forms.
Wasp provides sign-in buttons and URLs for each of the supported social login providers.
* JavaScript
* TypeScript
src/LoginPage.jsx
```
import { GoogleSignInButton, googleSignInUrl, GitHubSignInButton, gitHubSignInUrl,} from 'wasp/client/auth'export const LoginPage = () => { return ( <> <GoogleSignInButton /> <GitHubSignInButton /> {/* or */} <a href={googleSignInUrl}>Sign in with Google</a> <a href={gitHubSignInUrl}>Sign in with GitHub</a> </> )}
```
If you need even more customization, you can create your custom components using `signInUrl`s.
## API Reference[](#api-reference "Direct link to API Reference")
### Fields in the `app.auth` Dictionary and Overrides[](#fields-in-the-appauth-dictionary-and-overrides "Direct link to fields-in-the-appauth-dictionary-and-overrides")
For more information on:
* Allowed fields in `app.auth`
* `userSignupFields` and `configFn` functions
Check the provider-specific API References: | null | https://wasp-lang.dev/docs/auth/social-auth/overview | Social login options (e.g., Log in with Google) are a great (maybe even the best) solution for handling user accounts. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecd1918cb6fe6-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:04 GMT | Tue, 23 Apr 2024 15:24:04 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=5bBgnEVW9wUmJua33z%2FsNwM4odF4y3o2T0ppHyBTPUALIQ5IUagXmJ0CsoLVHBE%2FGDPb1UVGaYq%2BZEFhHhju4hJ3ZqhPPQ9AbktmZZHt5uoeSmv7i6CK9Qzu%2BN8GXlNx"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 032545ff354f1968a8c4e5411675cdf2d7399ec4 | null | 937A:83772:39907E5:44AD426:6627D03B | null | MISS | cache-iad-kiad7000124-IAD | S1713885244.358845,VS0,VE17 | null | null | en | og:image | https://wasp-lang.dev/docs/auth/social-auth/overview | og:url | Overview | Wasp | og:title | Social login options (e.g., Log in with Google) are a great (maybe even the best) solution for handling user accounts. | og:description | Overview | Wasp | null | Overview
Social login options (e.g., Log in with Google) are a great (maybe even the best) solution for handling user accounts. A famous old developer joke tells us "The best auth system is the one you never have to make."
Wasp wants to make adding social login options to your app as painless as possible.
Using different social providers gives users a chance to sign into your app via their existing accounts on other platforms (Google, GitHub, etc.).
This page goes through the common behaviors between all supported social login providers and shows you how to customize them. It also gives an overview of Wasp's UI helpers - the quickest possible way to get started with social auth.
Available Providers
Wasp currently supports the following social login providers:
User Entity
Wasp requires you to declare a userEntity for all auth methods (social or otherwise). This field tells Wasp which Entity represents the user.
Here's what the full setup looks like:
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
google: {}
},
onAuthFailedRedirectTo: "/login"
},
}
entity User {=psl
id Int @id @default(autoincrement())
//...
psl=}
To learn more about what the fields on these entities represent, look at the API Reference.
Default Behavior
When a user signs in for the first time, Wasp creates a new user account and links it to the chosen auth provider account for future logins.
Overrides
By default, Wasp doesn't store any information it receives from the social login provider. It only stores the user's ID specific to the provider.
If you wish to store more information about the user, you can override the default behavior. You can do this by defining the userSignupFields and configFn fields in main.wasp for each provider.
You can create custom signup setups, such as allowing users to define a custom username after they sign up with a social provider.
Example: Allowing User to Set Their Username
If you want to modify the signup flow (e.g., let users choose their own usernames), you will need to go through three steps:
The first step is adding a isSignupComplete property to your User Entity. This field will signal whether the user has completed the signup process.
The second step is overriding the default signup behavior.
The third step is implementing the rest of your signup flow and redirecting users where appropriate.
Let's go through both steps in more detail.
1. Adding the isSignupComplete Field to the User Entity
JavaScript
TypeScript
main.wasp
entity User {=psl
id Int @id @default(autoincrement())
username String? @unique
isSignupComplete Boolean @default(false)
psl=}
2. Overriding the Default Behavior
Declare an import under app.auth.methods.google.userSignupFields (the example assumes you're using Google):
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
google: {
userSignupFields: import { userSignupFields } from "@src/auth/google.js"
}
},
onAuthFailedRedirectTo: "/login"
},
}
// ...
And implement the imported function.
src/auth/google.js
export const userSignupFields = {
isSignupComplete: () => false,
}
3. Showing the Correct State on the Client
You can query the user's isSignupComplete flag on the client with the useAuth() hook. Depending on the flag's value, you can redirect users to the appropriate signup step.
For example:
When the user lands on the homepage, check the value of user.isSignupComplete.
If it's false, it means the user has started the signup process but hasn't yet chosen their username. Therefore, you can redirect them to EditUserDetailsPage where they can edit the username property.
JavaScript
TypeScript
src/HomePage.jsx
import { useAuth } from 'wasp/client/auth'
import { Redirect } from 'react-router-dom'
export function HomePage() {
const { data: user } = useAuth()
if (user.isSignupComplete === false) {
return <Redirect to="/edit-user-details" />
}
// ...
}
Using the User's Provider Account Details
Account details are provider-specific. Each provider has their own rules for defining the userSignupFields and configFn fields:
UI Helpers
Use Auth UI
Auth UI is a common name for all high-level auth forms that come with Wasp.
These include fully functional auto-generated login and signup forms with working social login buttons. If you're looking for the fastest way to get your auth up and running, that's where you should look.
The UI helpers described below are lower-level and are useful for creating your custom forms.
Wasp provides sign-in buttons and URLs for each of the supported social login providers.
JavaScript
TypeScript
src/LoginPage.jsx
import {
GoogleSignInButton,
googleSignInUrl,
GitHubSignInButton,
gitHubSignInUrl,
} from 'wasp/client/auth'
export const LoginPage = () => {
return (
<>
<GoogleSignInButton />
<GitHubSignInButton />
{/* or */}
<a href={googleSignInUrl}>Sign in with Google</a>
<a href={gitHubSignInUrl}>Sign in with GitHub</a>
</>
)
}
If you need even more customization, you can create your custom components using signInUrls.
API Reference
Fields in the app.auth Dictionary and Overrides
For more information on:
Allowed fields in app.auth
userSignupFields and configFn functions
Check the provider-specific API References: | https://wasp-lang.dev/docs/auth/social-auth/overview |
|
1 | null | 2024-04-23T15:14:08.715Z | https://wasp-lang.dev/docs/auth/social-auth/google | https://wasp-lang.dev/docs | http | ## Google
Wasp supports Google Authentication out of the box. Google Auth is arguably the best external auth option, as most users on the web already have Google accounts.
Enabling it lets your users log in using their existing Google accounts, greatly simplifying the process and enhancing the user experience.
Let's walk through enabling Google authentication, explain some of the default settings, and show how to override them.
## Setting up Google Auth[](#setting-up-google-auth "Direct link to Setting up Google Auth")
Enabling Google Authentication comes down to a series of steps:
1. Enabling Google authentication in the Wasp file.
2. Adding the `User` entity.
3. Creating a Google OAuth app.
4. Adding the necessary Routes and Pages
5. Using Auth UI components in our Pages.
Here's a skeleton of how our `main.wasp` should look like after we're done:
main.wasp
```
// Configuring the social authenticationapp myApp { auth: { ... }}// Defining entitiesentity User { ... }// Defining routes and pagesroute LoginRoute { ... }page LoginPage { ... }
```
### 1\. Adding Google Auth to Your Wasp File[](#1-adding-google-auth-to-your-wasp-file "Direct link to 1. Adding Google Auth to Your Wasp File")
Let's start by properly configuring the Auth object:
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { // 1. Specify the User entity (we'll define it next) userEntity: User, methods: { // 2. Enable Google Auth google: {} }, onAuthFailedRedirectTo: "/login" },}
```
`userEntity` is explained in [the social auth overview](https://wasp-lang.dev/docs/auth/social-auth/overview#social-login-entity).
### 2\. Adding the User Entity[](#2-adding-the-user-entity "Direct link to 2. Adding the User Entity")
Let's now define the `app.auth.userEntity` entity:
* JavaScript
* TypeScript
main.wasp
```
// ...// 3. Define the User entityentity User {=psl id Int @id @default(autoincrement()) // ...psl=}
```
### 3\. Creating a Google OAuth App[](#3-creating-a-google-oauth-app "Direct link to 3. Creating a Google OAuth App")
To use Google as an authentication method, you'll first need to create a Google project and provide Wasp with your client key and secret. Here's how you do it:
1. Create a Google Cloud Platform account if you do not already have one: [https://cloud.google.com/](https://cloud.google.com/)
2. Create and configure a new Google project here: [https://console.cloud.google.com/home/dashboard](https://console.cloud.google.com/home/dashboard)
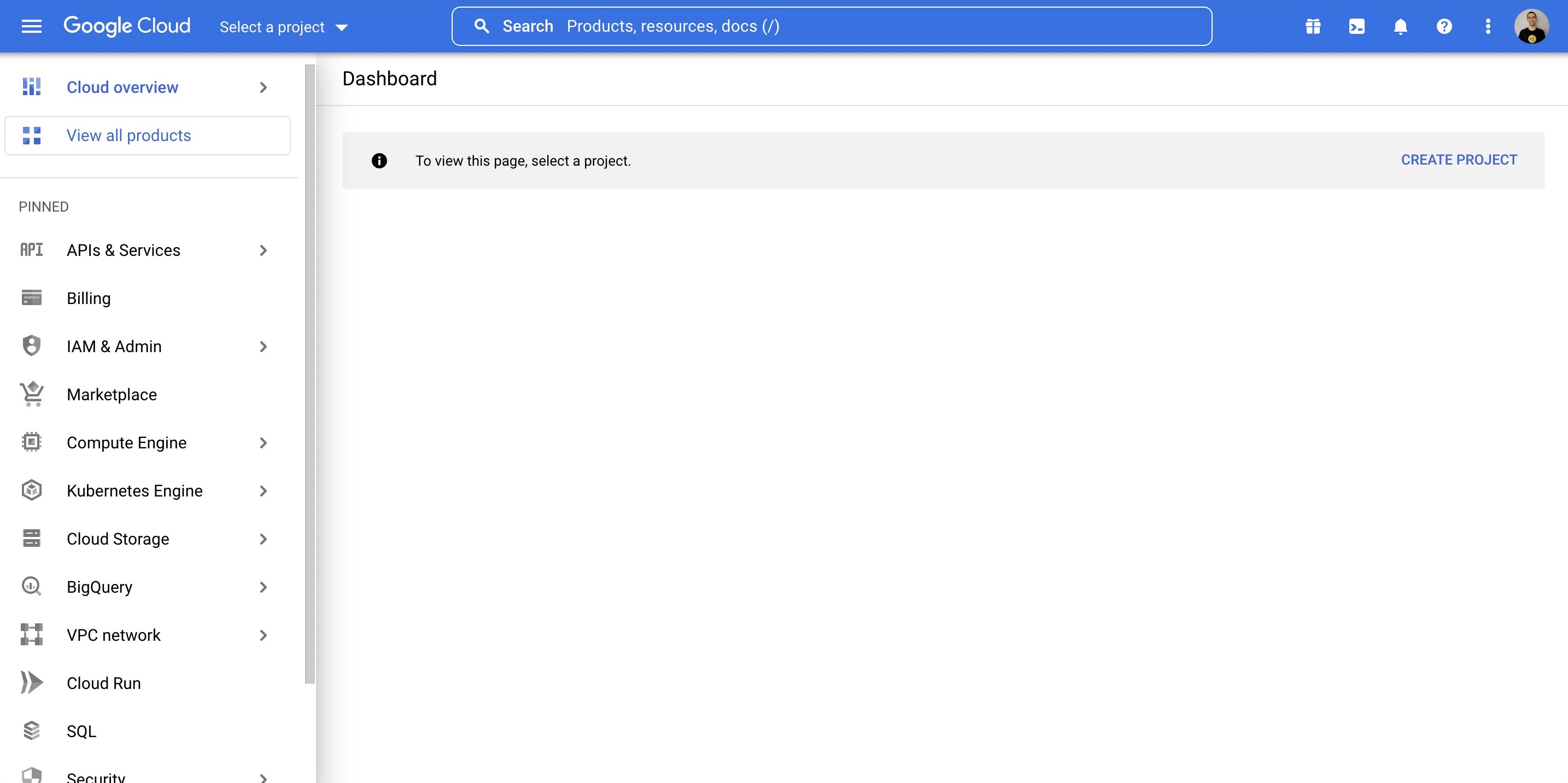
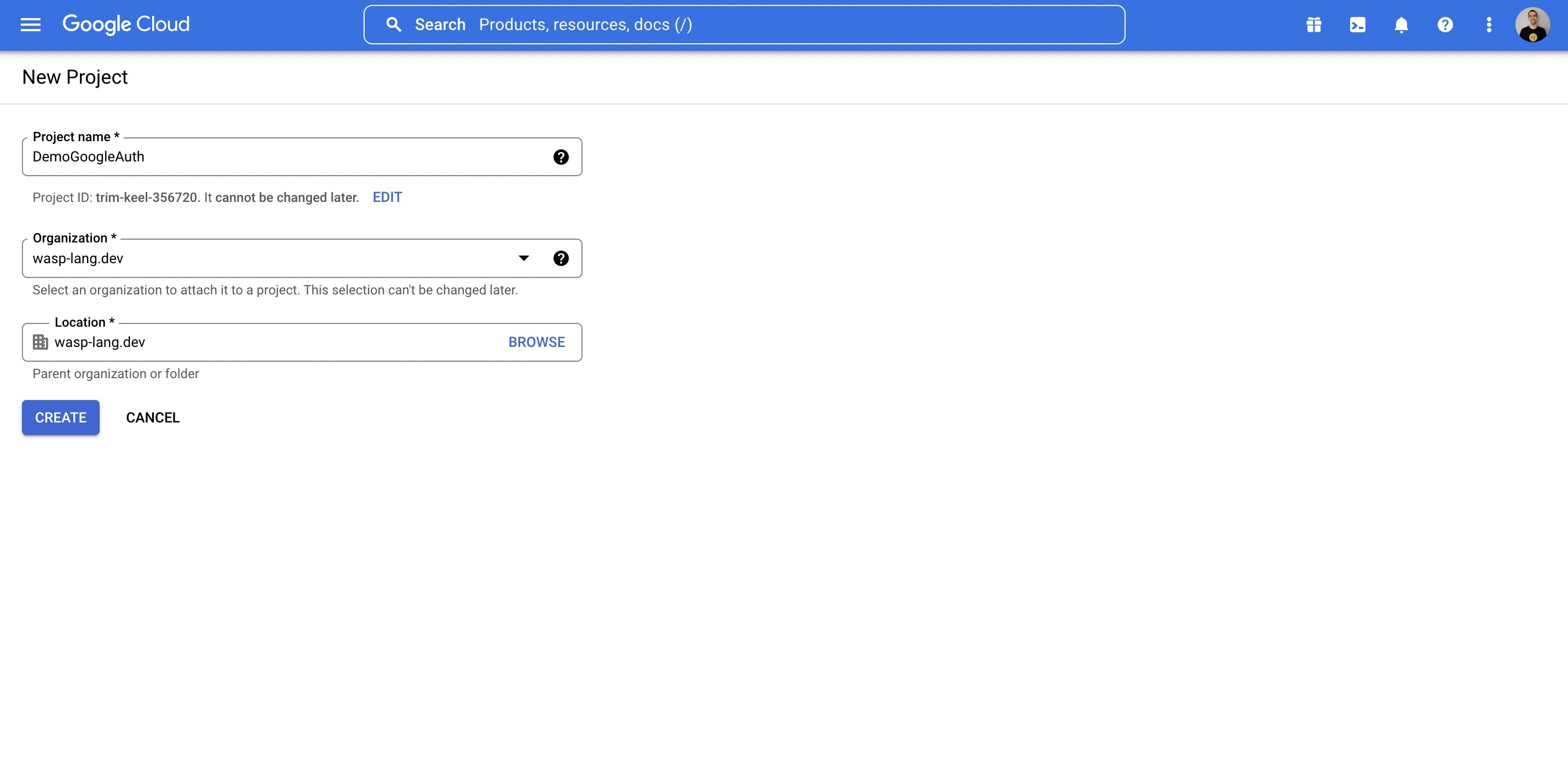
3. Search for **OAuth** in the top bar, click on **OAuth consent screen**.
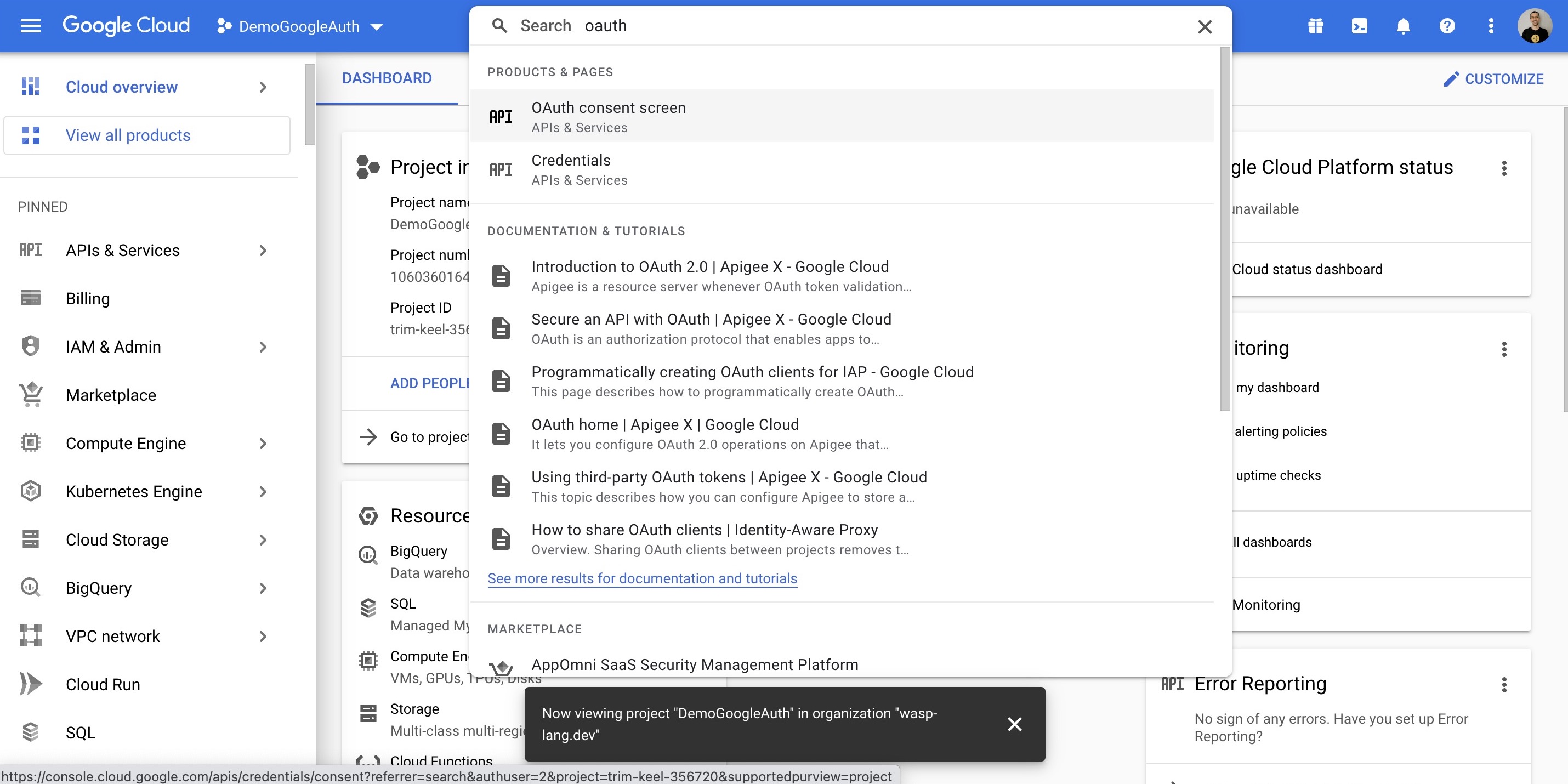
* Select what type of app you want, we will go with **External**.
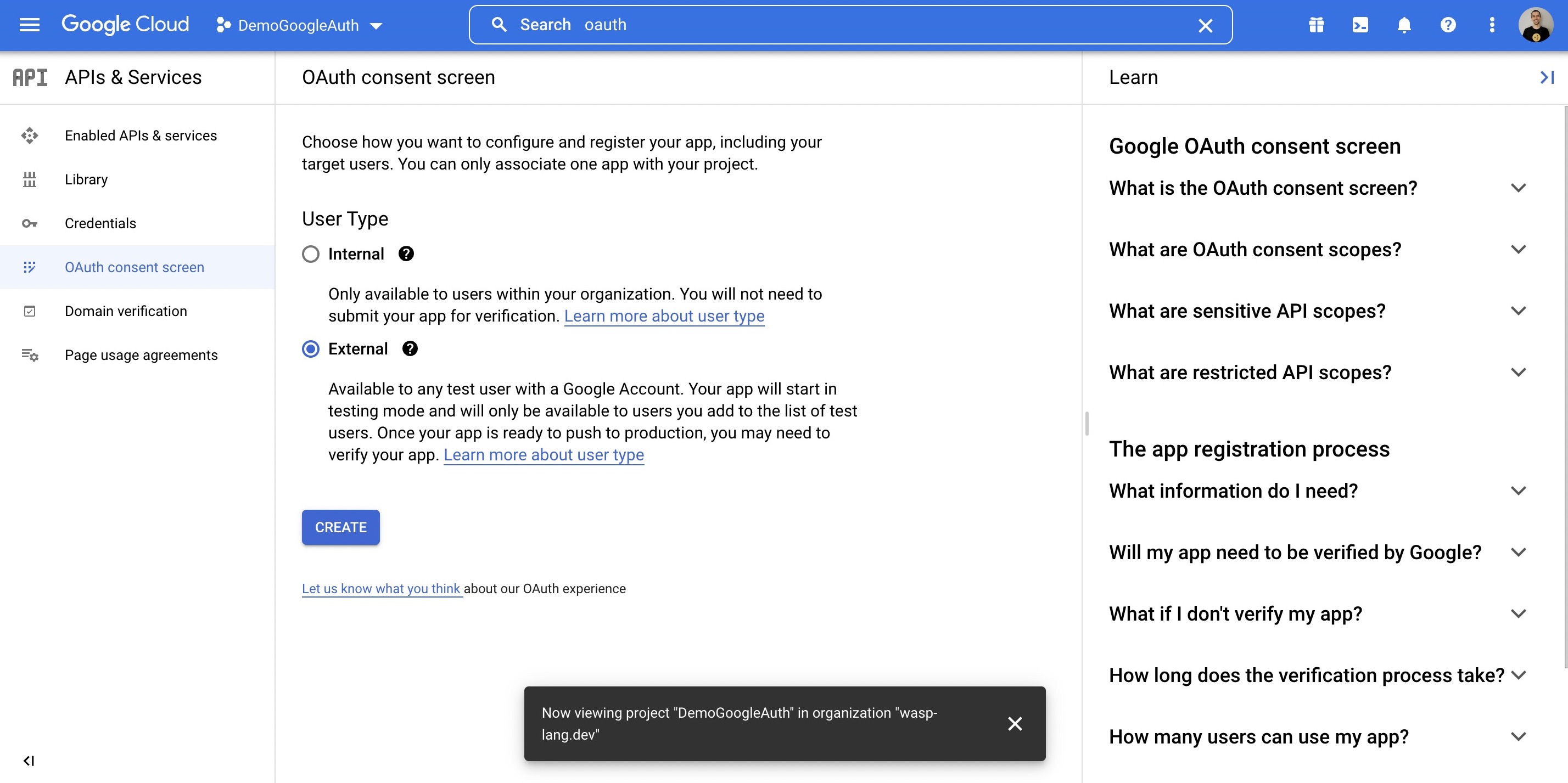
* Fill out applicable information on Page 1.
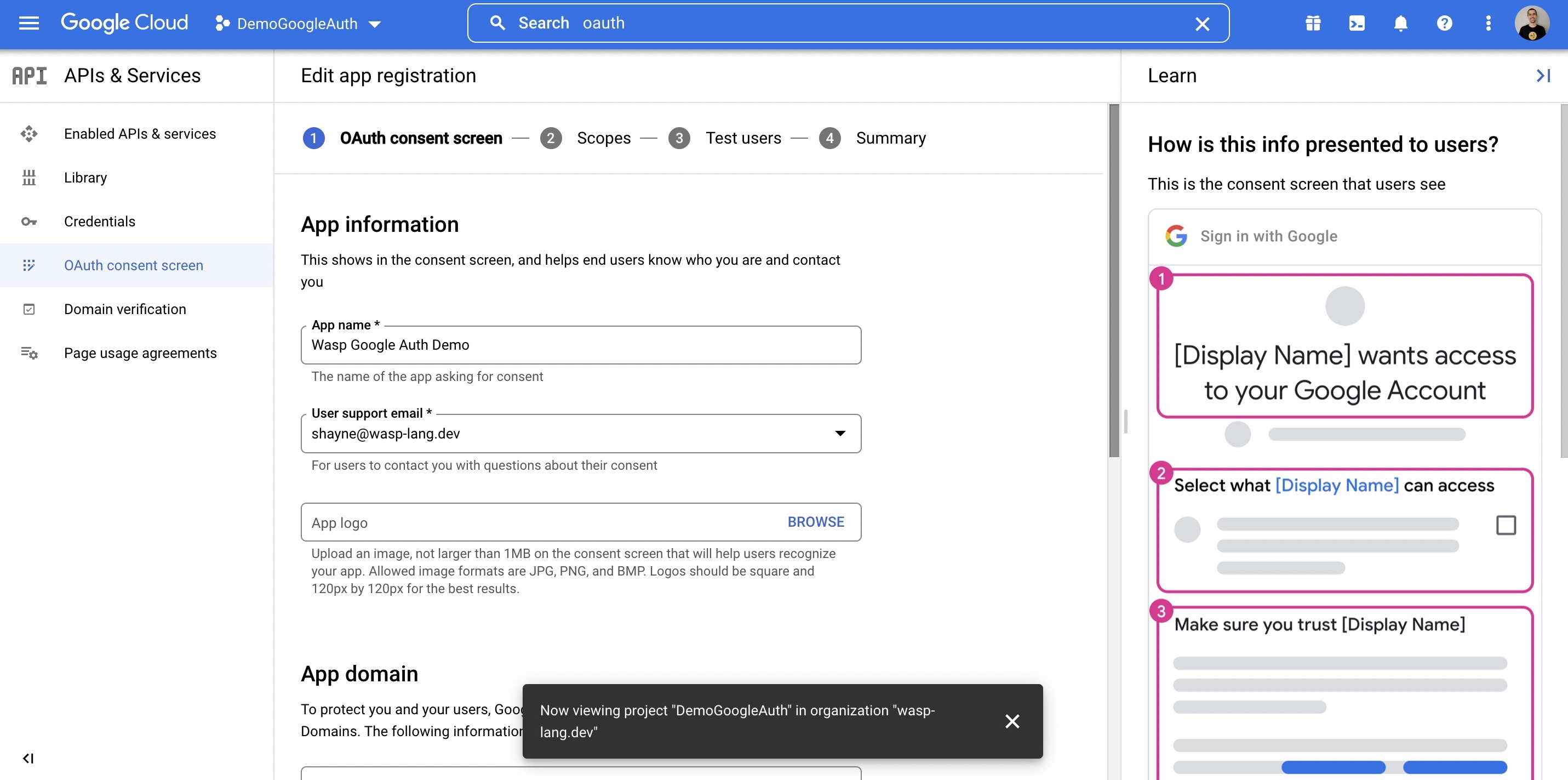
* On Page 2, Scopes, you should select `userinfo.profile`. You can optionally search for other things, like `email`.
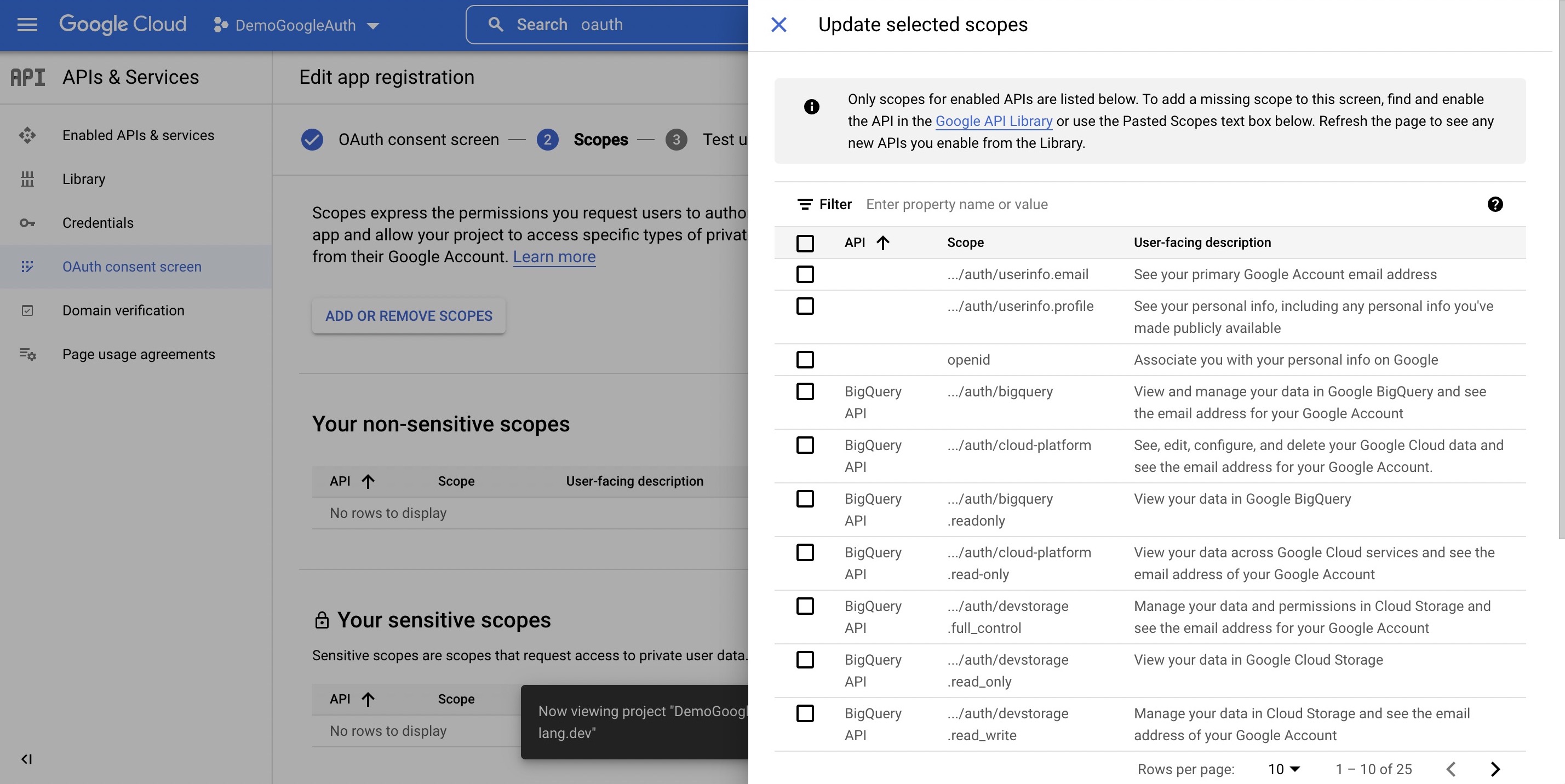
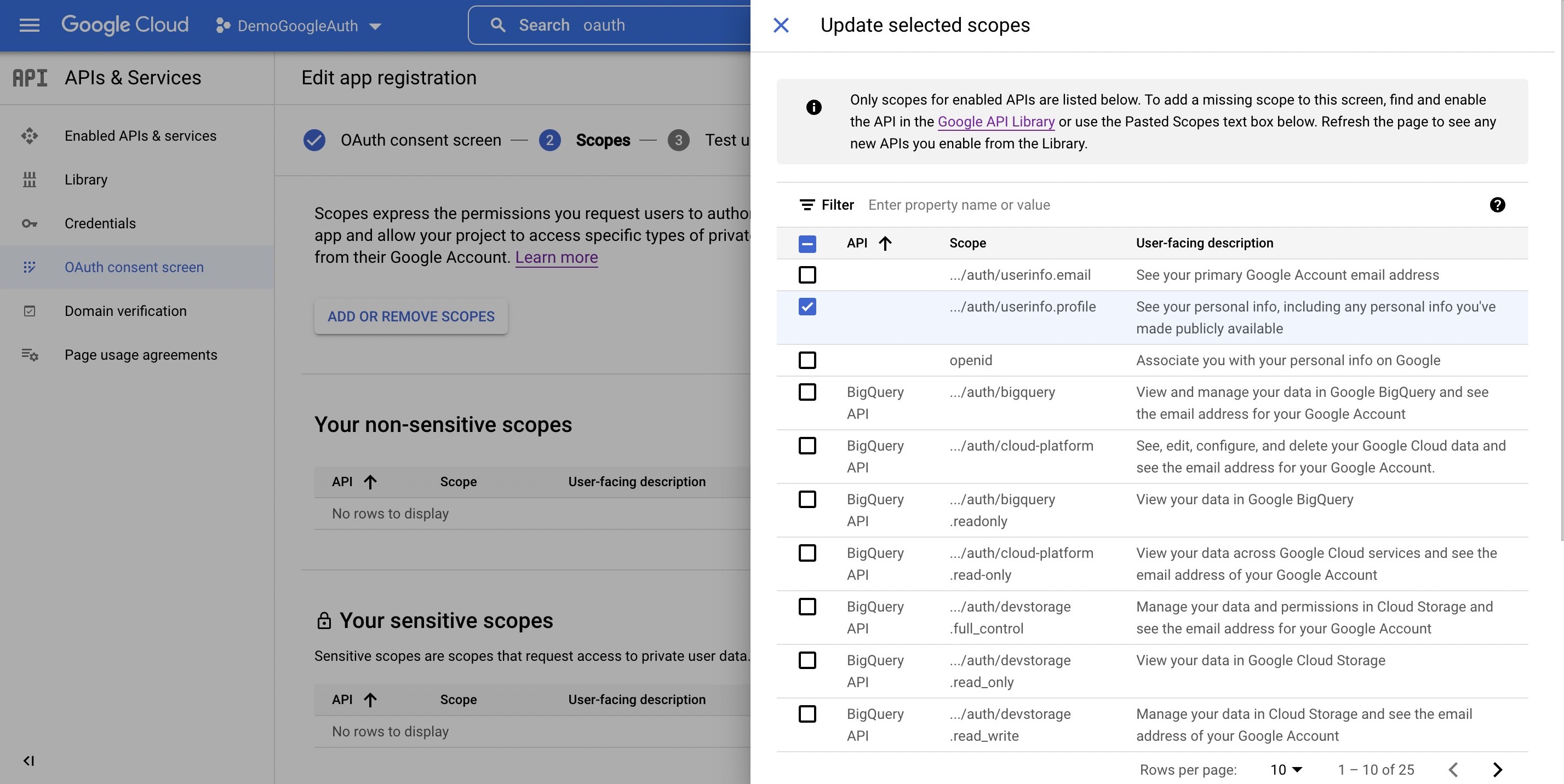
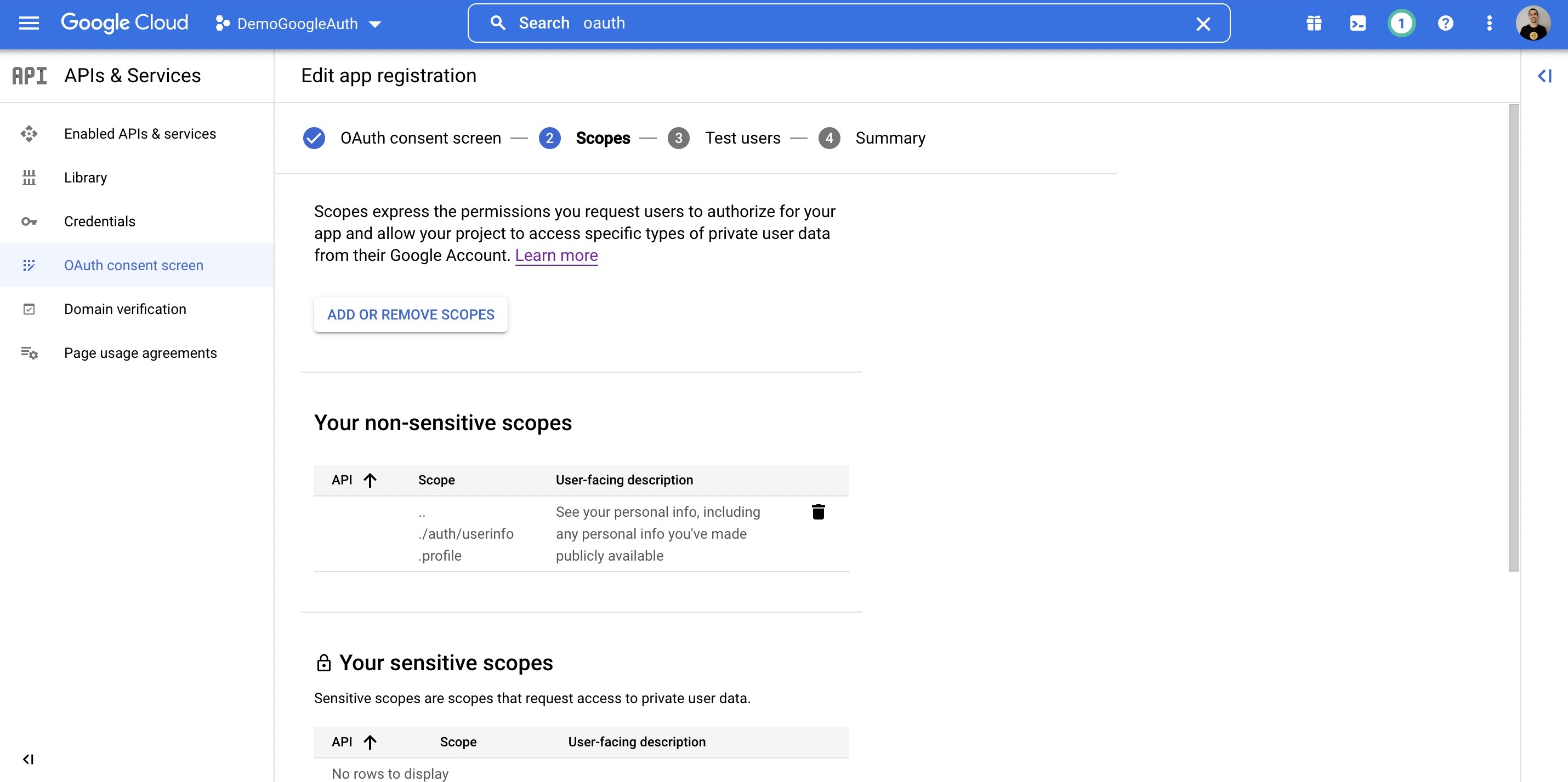
* Add any test users you want on Page 3.
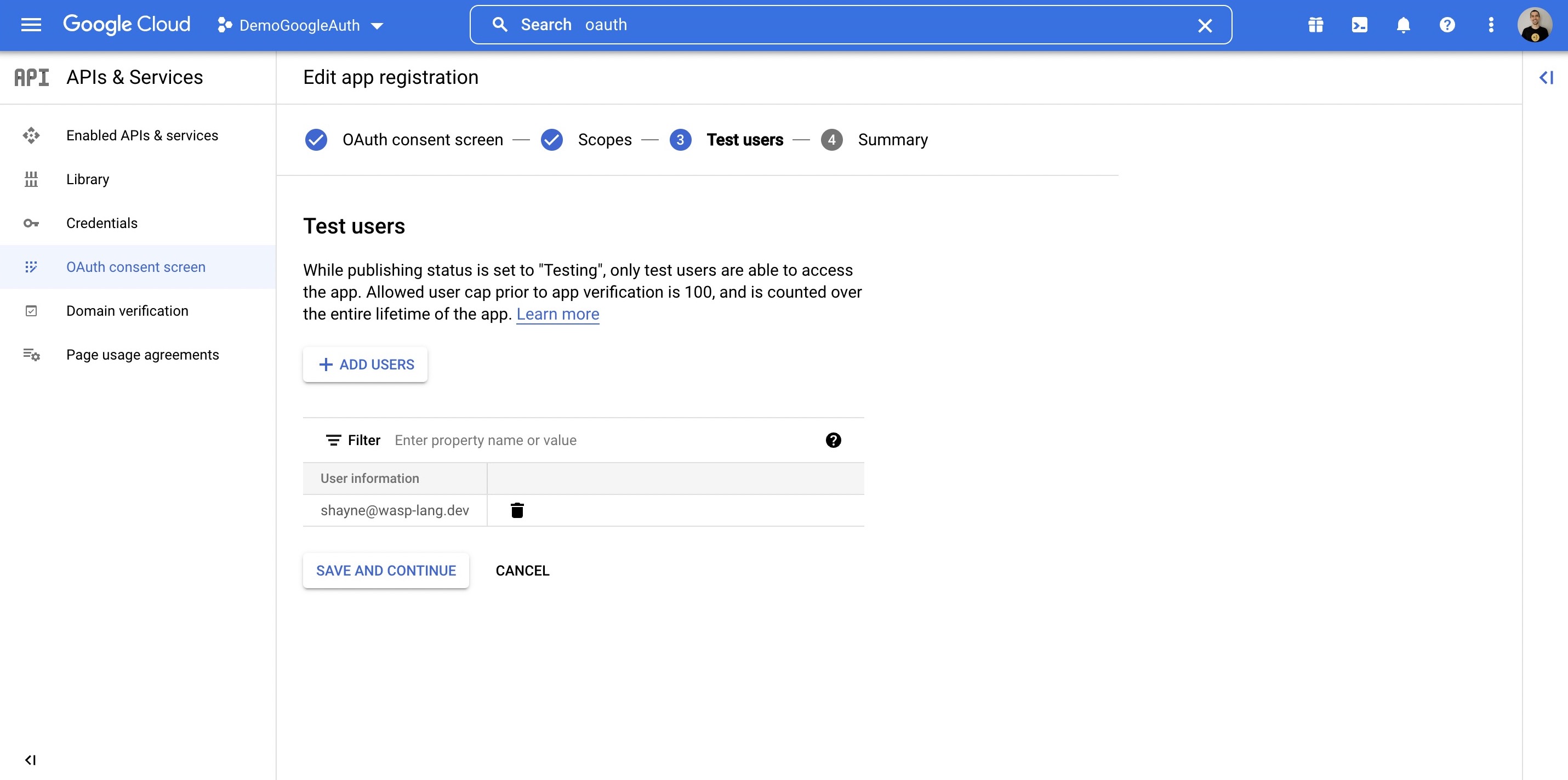
4. Next, click **Credentials**.
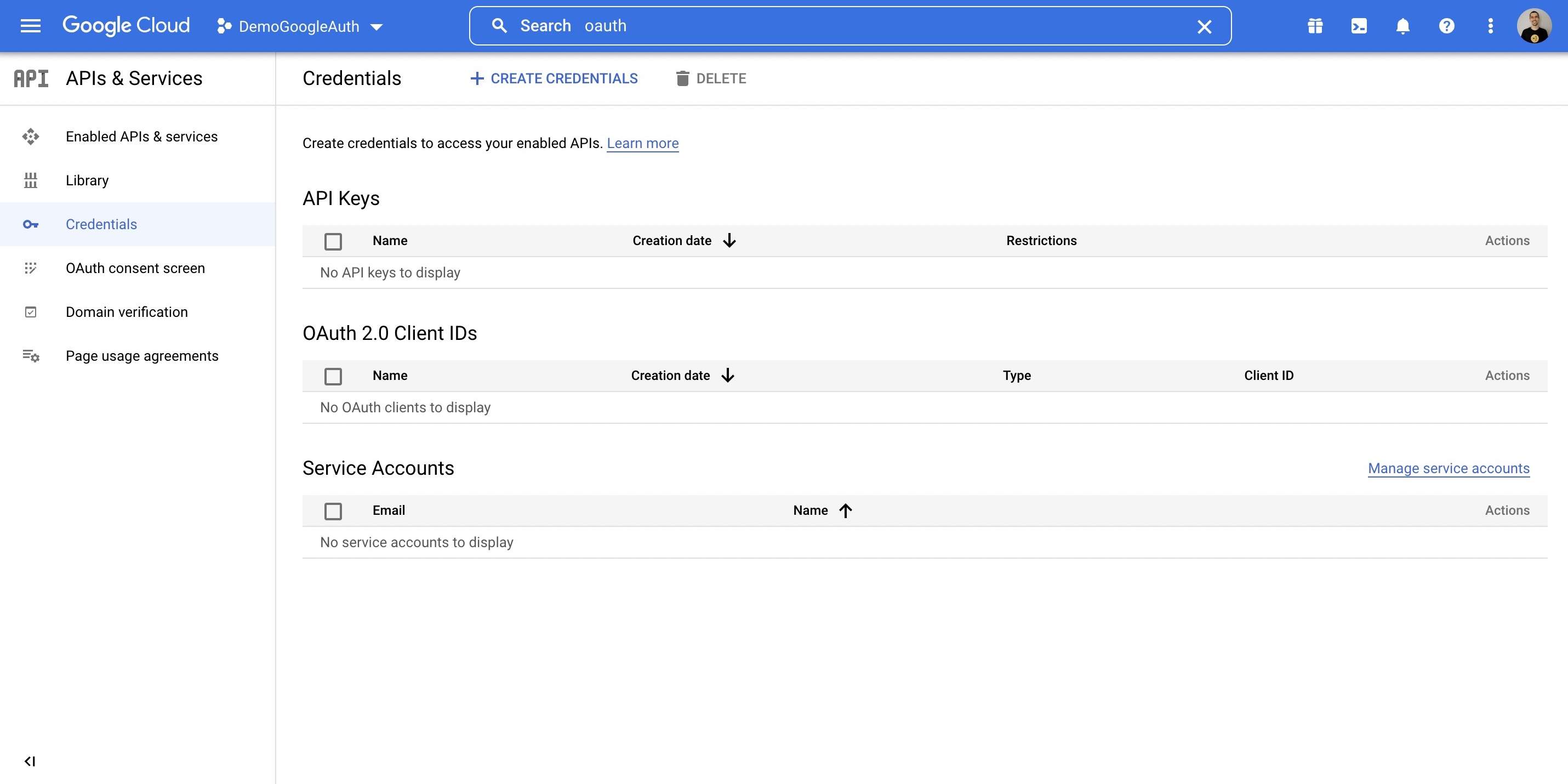
* Select **Create Credentials**.
* Select **OAuth client ID**.
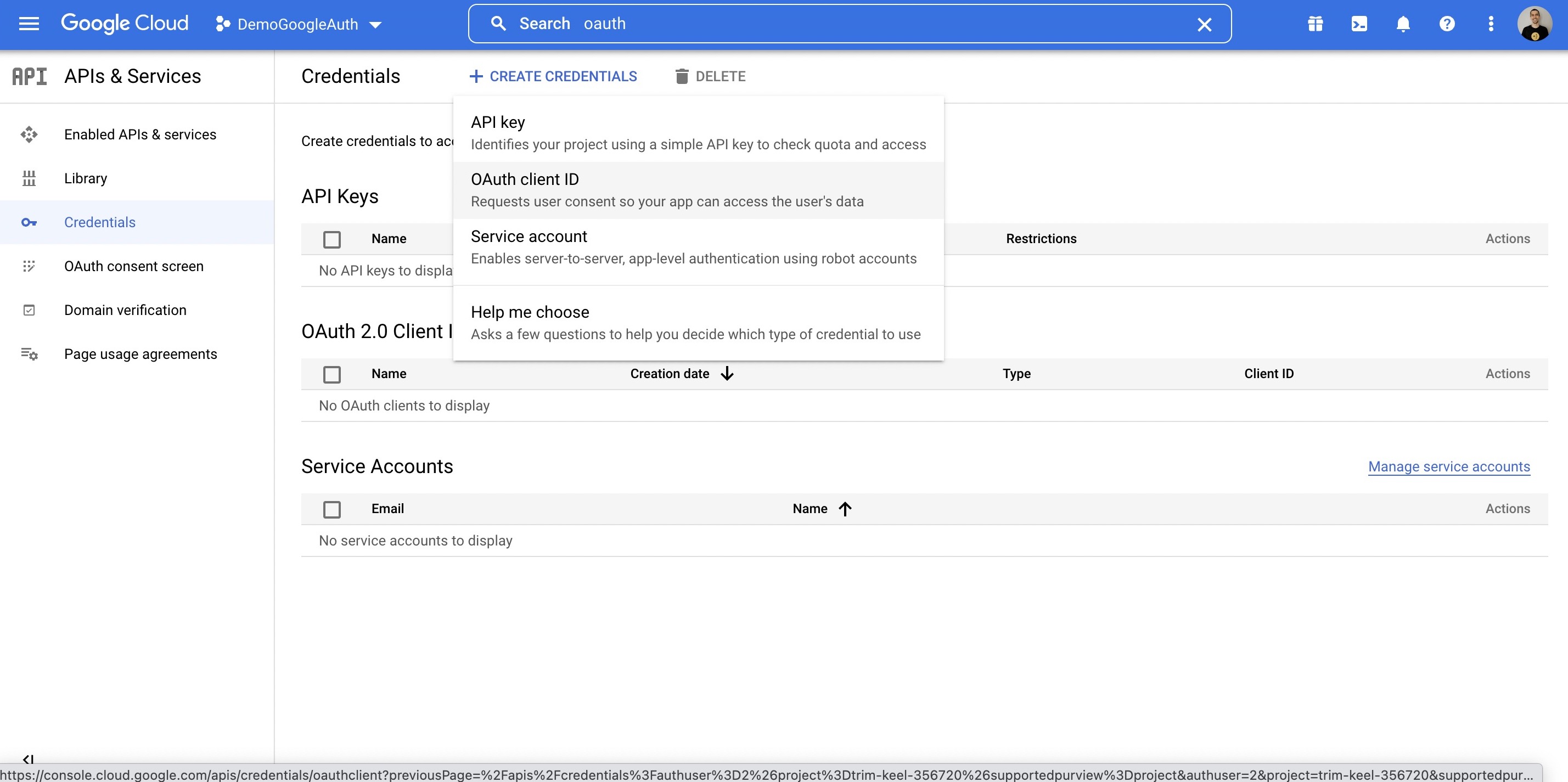
* Complete the form
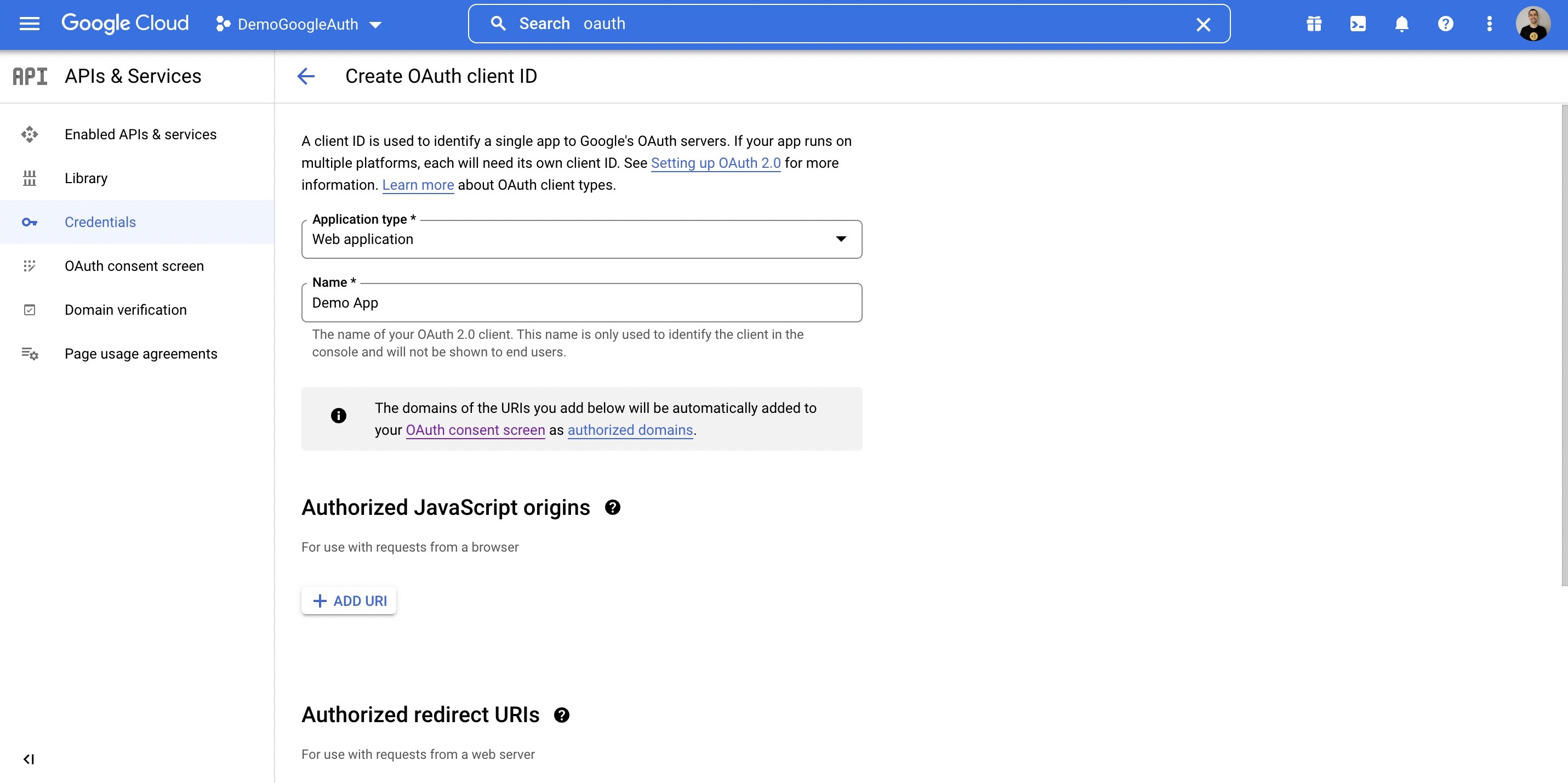
* Under Authorized redirect URIs, put in: `http://localhost:3001/auth/google/callback`
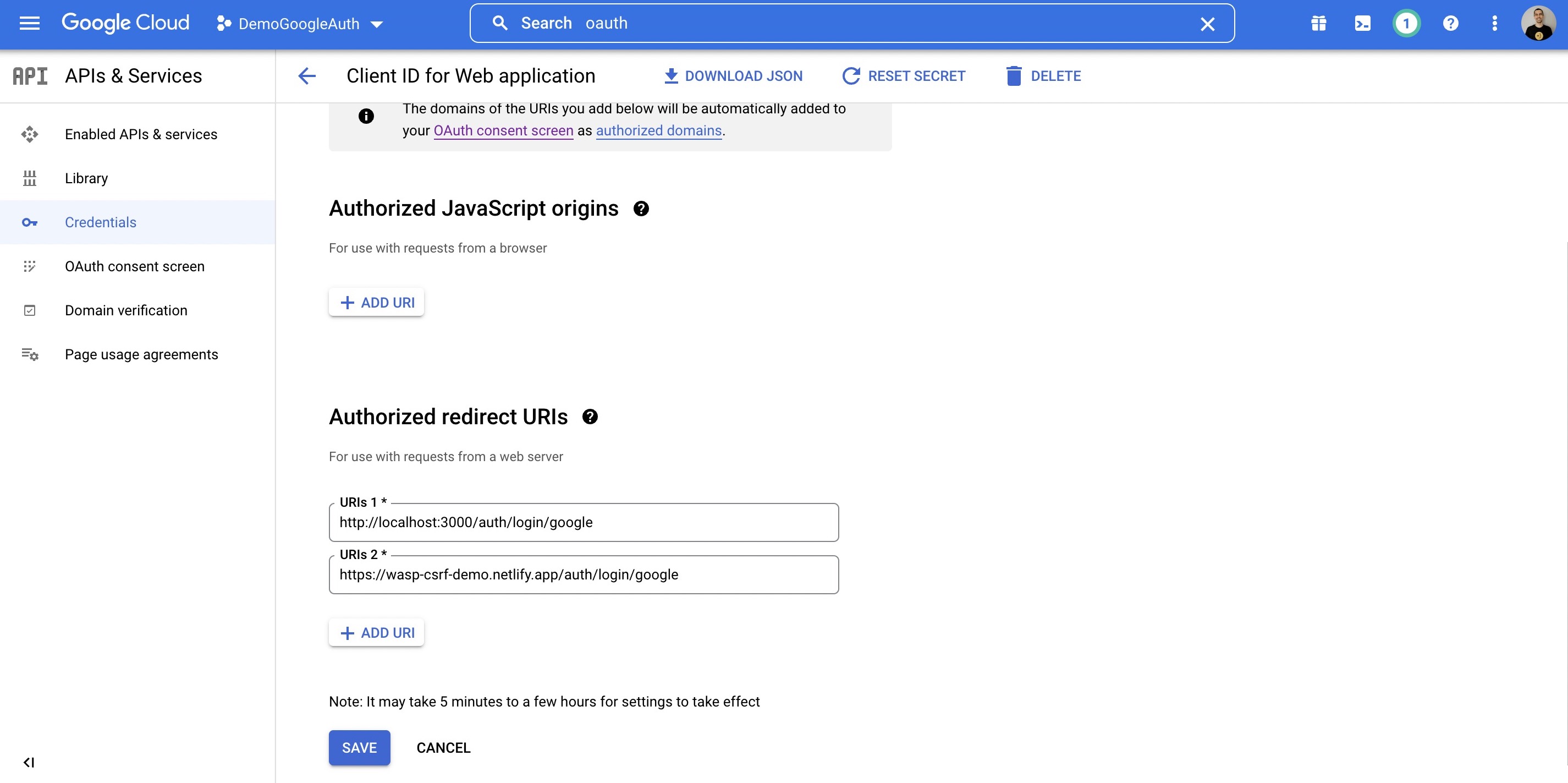
* Once you know on which URL(s) your API server will be deployed, also add those URL(s).
* For example: `https://your-server-url.com/auth/google/callback`
* When you save, you can click the Edit icon and your credentials will be shown.
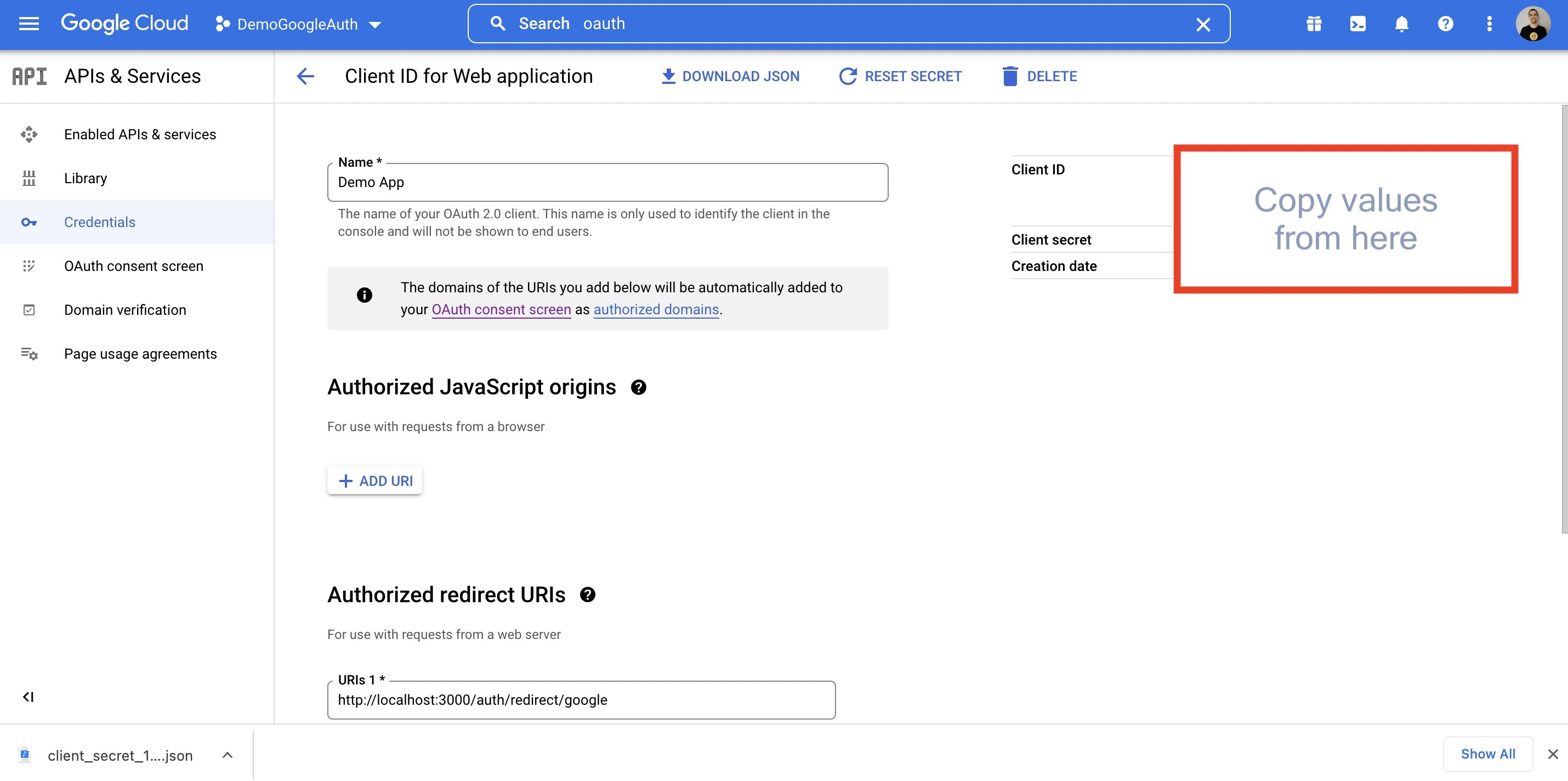
5. Copy your Client ID and Client secret as you will need them in the next step.
### 4\. Adding Environment Variables[](#4-adding-environment-variables "Direct link to 4. Adding Environment Variables")
Add these environment variables to the `.env.server` file at the root of your project (take their values from the previous step):
.env.server
```
GOOGLE_CLIENT_ID=your-google-client-idGOOGLE_CLIENT_SECRET=your-google-client-secret
```
### 5\. Adding the Necessary Routes and Pages[](#5-adding-the-necessary-routes-and-pages "Direct link to 5. Adding the Necessary Routes and Pages")
Let's define the necessary authentication Routes and Pages.
Add the following code to your `main.wasp` file:
* JavaScript
* TypeScript
main.wasp
```
// ...// 6. Define the routesroute LoginRoute { path: "/login", to: LoginPage }page LoginPage { component: import { Login } from "@src/pages/auth.jsx"}
```
We'll define the React components for these pages in the `src/pages/auth.tsx` file below.
### 6\. Create the Client Pages[](#6-create-the-client-pages "Direct link to 6. Create the Client Pages")
info
We are using [Tailwind CSS](https://tailwindcss.com/) to style the pages. Read more about how to add it [here](https://wasp-lang.dev/docs/project/css-frameworks).
Let's now create a `auth.tsx` file in the `src/pages`. It should have the following code:
* JavaScript
* TypeScript
src/pages/auth.jsx
```
import { LoginForm } from 'wasp/client/auth'export function Login() { return ( <Layout> <LoginForm /> </Layout> )}// A layout component to center the contentexport function Layout({ children }) { return ( <div className="w-full h-full bg-white"> <div className="min-w-full min-h-[75vh] flex items-center justify-center"> <div className="w-full h-full max-w-sm p-5 bg-white"> <div>{children}</div> </div> </div> </div> )}
```
Auth UI
Our pages use an automatically-generated Auth UI component. Read more about Auth UI components [here](https://wasp-lang.dev/docs/auth/ui).
### Conclusion[](#conclusion "Direct link to Conclusion")
Yay, we've successfully set up Google Auth! 🎉
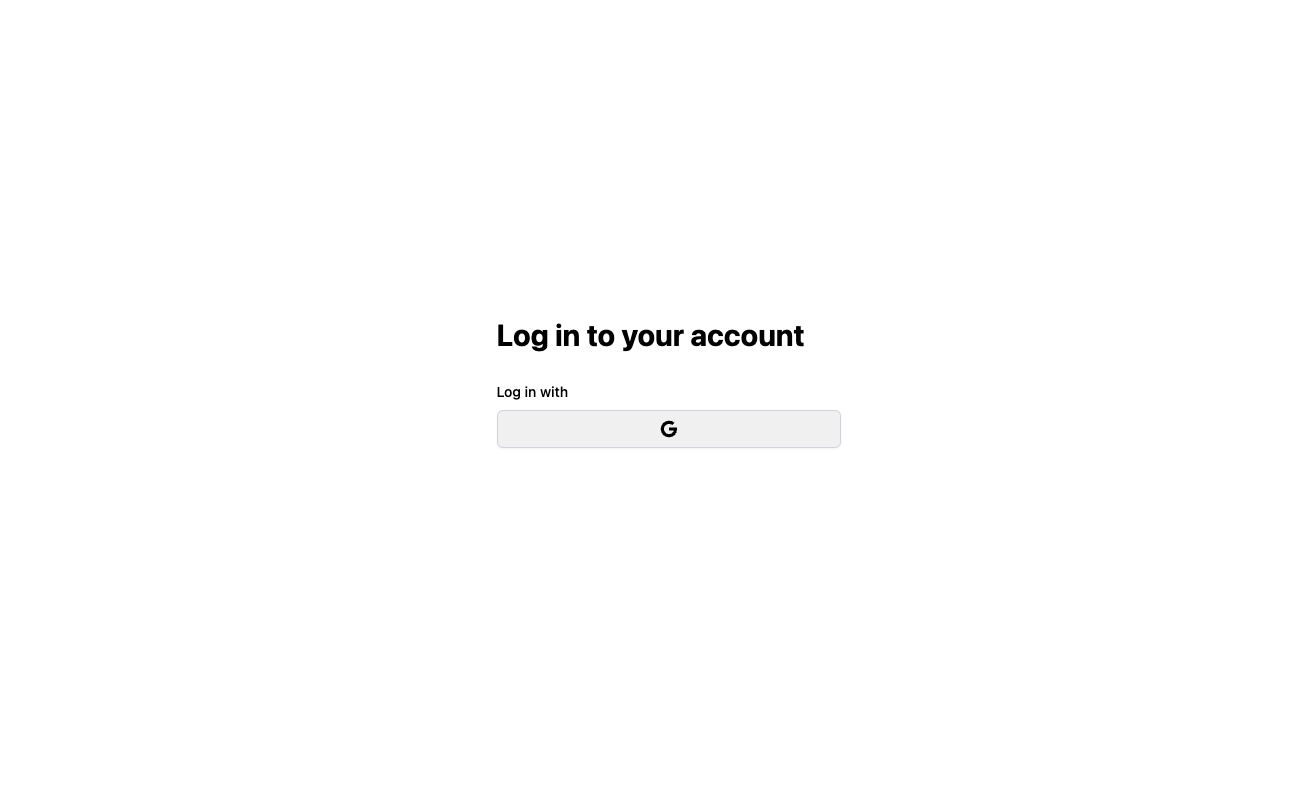
Running `wasp db migrate-dev` and `wasp start` should now give you a working app with authentication. To see how to protect specific pages (i.e., hide them from non-authenticated users), read the docs on [using auth](https://wasp-lang.dev/docs/auth/overview).
## Default Behaviour[](#default-behaviour "Direct link to Default Behaviour")
Add `google: {}` to the `auth.methods` dictionary to use it with default settings:
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { google: {} }, onAuthFailedRedirectTo: "/login" },}
```
When a user **signs in for the first time**, Wasp creates a new user account and links it to the chosen auth provider account for future logins.
## Overrides[](#overrides "Direct link to Overrides")
By default, Wasp doesn't store any information it receives from the social login provider. It only stores the user's ID specific to the provider.
There are two mechanisms used for overriding the default behavior:
* `userSignupFields`
* `configFn`
Let's explore them in more detail.
### Data Received From Google[](#data-received-from-google "Direct link to Data Received From Google")
We are using Google's API and its `/userinfo` endpoint to fetch the user's data.
The data received from Google is an object which can contain the following fields:
```
[ "name", "given_name", "family_name", "email", "email_verified", "aud", "exp", "iat", "iss", "locale", "picture", "sub"]
```
The fields you receive depend on the scopes you request. The default scope is set to `profile` only. If you want to get the user's email, you need to specify the `email` scope in the `configFn` function.
For an up to date info about the data received from Google, please refer to the [Google API documentation](https://developers.google.com/identity/openid-connect/openid-connect#an-id-tokens-payload).
### Using the Data Received From Google[](#using-the-data-received-from-google "Direct link to Using the Data Received From Google")
When a user logs in using a social login provider, the backend receives some data about the user. Wasp lets you access this data inside the `userSignupFields` getters.
For example, the User entity can include a `displayName` field which you can set based on the details received from the provider.
Wasp also lets you customize the configuration of the providers' settings using the `configFn` function.
Let's use this example to show both fields in action:
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { google: { configFn: import { getConfig } from "@src/auth/google.js", userSignupFields: import { userSignupFields } from "@src/auth/google.js" } }, onAuthFailedRedirectTo: "/login" },}entity User {=psl id Int @id @default(autoincrement()) username String @unique displayName Stringpsl=}// ...
```
src/auth/google.js
```
export const userSignupFields = { username: () => "hardcoded-username", displayName: (data) => data.profile.name,}export function getConfig() { return { scopes: ['profile', 'email'], }}
```
## Using Auth[](#using-auth "Direct link to Using Auth")
To read more about how to set up the logout button and get access to the logged-in user in both client and server code, read the docs on [using auth](https://wasp-lang.dev/docs/auth/overview).
## API Reference[](#api-reference "Direct link to API Reference")
Provider-specific behavior comes down to implementing two functions.
* `configFn`
* `userSignupFields`
The reference shows how to define both.
For behavior common to all providers, check the general [API Reference](https://wasp-lang.dev/docs/auth/overview#api-reference).
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { google: { configFn: import { getConfig } from "@src/auth/google.js", userSignupFields: import { userSignupFields } from "@src/auth/google.js" } }, onAuthFailedRedirectTo: "/login" },}
```
The `google` dict has the following properties:
* #### `configFn: ExtImport`[](#configfn-extimport "Direct link to configfn-extimport")
This function must return an object with the scopes for the OAuth provider.
* JavaScript
* TypeScript
src/auth/google.js
```
export function getConfig() { return { scopes: ['profile', 'email'], }}
```
* #### `userSignupFields: ExtImport`[](#usersignupfields-extimport "Direct link to usersignupfields-extimport")
`userSignupFields` defines all the extra fields that need to be set on the `User` during the sign-up process. For example, if you have `address` and `phone` fields on your `User` entity, you can set them by defining the `userSignupFields` like this:
* JavaScript
* TypeScript
src/auth.js
```
import { defineUserSignupFields } from 'wasp/server/auth'export const userSignupFields = defineUserSignupFields({ address: (data) => { if (!data.address) { throw new Error('Address is required') } return data.address } phone: (data) => data.phone,})
```
Read more about the `userSignupFields` function [here](https://wasp-lang.dev/docs/auth/overview#1-defining-extra-fields). | null | https://wasp-lang.dev/docs/auth/social-auth/google | Wasp supports Google Authentication out of the box. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecd3399827fb2-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:08 GMT | Tue, 23 Apr 2024 15:24:08 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=CTmDyHgQspUTbOFP4M6O6LjEjv1bLqeCDzjo6tMo1evPba0feHSgcqHjP%2FAdjJ4V7rsgdqM8HJ2VmXBbf5NKfgpTgo%2FmKPqb9L9TuCflF1%2FMLhl51aLkKEpfMV1uYjUG"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 302071ee8ef4c438e91f7a311c4c23ef9a21b595 | null | 6154:2C39F4:3B2ABE2:4646C95:6627D03F | HIT | MISS | cache-iad-kiad7000029-IAD | S1713885249.608864,VS0,VE17 | null | null | en | og:image | https://wasp-lang.dev/docs/auth/social-auth/google | og:url | Google | Wasp | og:title | Wasp supports Google Authentication out of the box. | og:description | Google | Wasp | null | Google
Wasp supports Google Authentication out of the box. Google Auth is arguably the best external auth option, as most users on the web already have Google accounts.
Enabling it lets your users log in using their existing Google accounts, greatly simplifying the process and enhancing the user experience.
Let's walk through enabling Google authentication, explain some of the default settings, and show how to override them.
Setting up Google Auth
Enabling Google Authentication comes down to a series of steps:
Enabling Google authentication in the Wasp file.
Adding the User entity.
Creating a Google OAuth app.
Adding the necessary Routes and Pages
Using Auth UI components in our Pages.
Here's a skeleton of how our main.wasp should look like after we're done:
main.wasp
// Configuring the social authentication
app myApp {
auth: { ... }
}
// Defining entities
entity User { ... }
// Defining routes and pages
route LoginRoute { ... }
page LoginPage { ... }
1. Adding Google Auth to Your Wasp File
Let's start by properly configuring the Auth object:
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
// 1. Specify the User entity (we'll define it next)
userEntity: User,
methods: {
// 2. Enable Google Auth
google: {}
},
onAuthFailedRedirectTo: "/login"
},
}
userEntity is explained in the social auth overview.
2. Adding the User Entity
Let's now define the app.auth.userEntity entity:
JavaScript
TypeScript
main.wasp
// ...
// 3. Define the User entity
entity User {=psl
id Int @id @default(autoincrement())
// ...
psl=}
3. Creating a Google OAuth App
To use Google as an authentication method, you'll first need to create a Google project and provide Wasp with your client key and secret. Here's how you do it:
Create a Google Cloud Platform account if you do not already have one: https://cloud.google.com/
Create and configure a new Google project here: https://console.cloud.google.com/home/dashboard
Search for OAuth in the top bar, click on OAuth consent screen.
Select what type of app you want, we will go with External.
Fill out applicable information on Page 1.
On Page 2, Scopes, you should select userinfo.profile. You can optionally search for other things, like email.
Add any test users you want on Page 3.
Next, click Credentials.
Select Create Credentials.
Select OAuth client ID.
Complete the form
Under Authorized redirect URIs, put in: http://localhost:3001/auth/google/callback
Once you know on which URL(s) your API server will be deployed, also add those URL(s).
For example: https://your-server-url.com/auth/google/callback
When you save, you can click the Edit icon and your credentials will be shown.
Copy your Client ID and Client secret as you will need them in the next step.
4. Adding Environment Variables
Add these environment variables to the .env.server file at the root of your project (take their values from the previous step):
.env.server
GOOGLE_CLIENT_ID=your-google-client-id
GOOGLE_CLIENT_SECRET=your-google-client-secret
5. Adding the Necessary Routes and Pages
Let's define the necessary authentication Routes and Pages.
Add the following code to your main.wasp file:
JavaScript
TypeScript
main.wasp
// ...
// 6. Define the routes
route LoginRoute { path: "/login", to: LoginPage }
page LoginPage {
component: import { Login } from "@src/pages/auth.jsx"
}
We'll define the React components for these pages in the src/pages/auth.tsx file below.
6. Create the Client Pages
info
We are using Tailwind CSS to style the pages. Read more about how to add it here.
Let's now create a auth.tsx file in the src/pages. It should have the following code:
JavaScript
TypeScript
src/pages/auth.jsx
import { LoginForm } from 'wasp/client/auth'
export function Login() {
return (
<Layout>
<LoginForm />
</Layout>
)
}
// A layout component to center the content
export function Layout({ children }) {
return (
<div className="w-full h-full bg-white">
<div className="min-w-full min-h-[75vh] flex items-center justify-center">
<div className="w-full h-full max-w-sm p-5 bg-white">
<div>{children}</div>
</div>
</div>
</div>
)
}
Auth UI
Our pages use an automatically-generated Auth UI component. Read more about Auth UI components here.
Conclusion
Yay, we've successfully set up Google Auth! 🎉
Running wasp db migrate-dev and wasp start should now give you a working app with authentication. To see how to protect specific pages (i.e., hide them from non-authenticated users), read the docs on using auth.
Default Behaviour
Add google: {} to the auth.methods dictionary to use it with default settings:
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
google: {}
},
onAuthFailedRedirectTo: "/login"
},
}
When a user signs in for the first time, Wasp creates a new user account and links it to the chosen auth provider account for future logins.
Overrides
By default, Wasp doesn't store any information it receives from the social login provider. It only stores the user's ID specific to the provider.
There are two mechanisms used for overriding the default behavior:
userSignupFields
configFn
Let's explore them in more detail.
Data Received From Google
We are using Google's API and its /userinfo endpoint to fetch the user's data.
The data received from Google is an object which can contain the following fields:
[
"name",
"given_name",
"family_name",
"email",
"email_verified",
"aud",
"exp",
"iat",
"iss",
"locale",
"picture",
"sub"
]
The fields you receive depend on the scopes you request. The default scope is set to profile only. If you want to get the user's email, you need to specify the email scope in the configFn function.
For an up to date info about the data received from Google, please refer to the Google API documentation.
Using the Data Received From Google
When a user logs in using a social login provider, the backend receives some data about the user. Wasp lets you access this data inside the userSignupFields getters.
For example, the User entity can include a displayName field which you can set based on the details received from the provider.
Wasp also lets you customize the configuration of the providers' settings using the configFn function.
Let's use this example to show both fields in action:
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
google: {
configFn: import { getConfig } from "@src/auth/google.js",
userSignupFields: import { userSignupFields } from "@src/auth/google.js"
}
},
onAuthFailedRedirectTo: "/login"
},
}
entity User {=psl
id Int @id @default(autoincrement())
username String @unique
displayName String
psl=}
// ...
src/auth/google.js
export const userSignupFields = {
username: () => "hardcoded-username",
displayName: (data) => data.profile.name,
}
export function getConfig() {
return {
scopes: ['profile', 'email'],
}
}
Using Auth
To read more about how to set up the logout button and get access to the logged-in user in both client and server code, read the docs on using auth.
API Reference
Provider-specific behavior comes down to implementing two functions.
configFn
userSignupFields
The reference shows how to define both.
For behavior common to all providers, check the general API Reference.
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
google: {
configFn: import { getConfig } from "@src/auth/google.js",
userSignupFields: import { userSignupFields } from "@src/auth/google.js"
}
},
onAuthFailedRedirectTo: "/login"
},
}
The google dict has the following properties:
configFn: ExtImport
This function must return an object with the scopes for the OAuth provider.
JavaScript
TypeScript
src/auth/google.js
export function getConfig() {
return {
scopes: ['profile', 'email'],
}
}
userSignupFields: ExtImport
userSignupFields defines all the extra fields that need to be set on the User during the sign-up process. For example, if you have address and phone fields on your User entity, you can set them by defining the userSignupFields like this:
JavaScript
TypeScript
src/auth.js
import { defineUserSignupFields } from 'wasp/server/auth'
export const userSignupFields = defineUserSignupFields({
address: (data) => {
if (!data.address) {
throw new Error('Address is required')
}
return data.address
}
phone: (data) => data.phone,
})
Read more about the userSignupFields function here. | https://wasp-lang.dev/docs/auth/social-auth/google |
|
1 | 200 | 2024-04-23T15:14:09.907Z | https://wasp-lang.dev/docs/auth/social-auth/github | https://wasp-lang.dev/docs | browser | ## GitHub
Wasp supports Github Authentication out of the box. GitHub is a great external auth choice when you're building apps for developers, as most of them already have a GitHub account.
Letting your users log in using their GitHub accounts turns the signup process into a breeze.
Let's walk through enabling Github Authentication, explain some of the default settings, and show how to override them.
## Setting up Github Auth[](#setting-up-github-auth "Direct link to Setting up Github Auth")
Enabling GitHub Authentication comes down to a series of steps:
1. Enabling GitHub authentication in the Wasp file.
2. Adding the `User` entity.
3. Creating a GitHub OAuth app.
4. Adding the necessary Routes and Pages
5. Using Auth UI components in our Pages.
Here's a skeleton of how our `main.wasp` should look like after we're done:
main.wasp
```
// Configuring the social authenticationapp myApp { auth: { ... }}// Defining entitiesentity User { ... }// Defining routes and pagesroute LoginRoute { ... }page LoginPage { ... }
```
### 1\. Adding Github Auth to Your Wasp File[](#1-adding-github-auth-to-your-wasp-file "Direct link to 1. Adding Github Auth to Your Wasp File")
Let's start by properly configuring the Auth object:
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { // 1. Specify the User entity (we'll define it next) userEntity: User, methods: { // 2. Enable Github Auth gitHub: {} }, onAuthFailedRedirectTo: "/login" },}
```
### 2\. Add the User Entity[](#2-add-the-user-entity "Direct link to 2. Add the User Entity")
Let's now define the `app.auth.userEntity` entity:
* JavaScript
* TypeScript
main.wasp
```
// ...// 3. Define the User entityentity User {=psl id Int @id @default(autoincrement()) // ...psl=}
```
### 3\. Creating a GitHub OAuth App[](#3-creating-a-github-oauth-app "Direct link to 3. Creating a GitHub OAuth App")
To use GitHub as an authentication method, you'll first need to create a GitHub OAuth App and provide Wasp with your client key and secret. Here's how you do it:
1. Log into your GitHub account and navigate to: [https://github.com/settings/developers](https://github.com/settings/developers).
2. Select **New OAuth App**.
3. Supply required information.
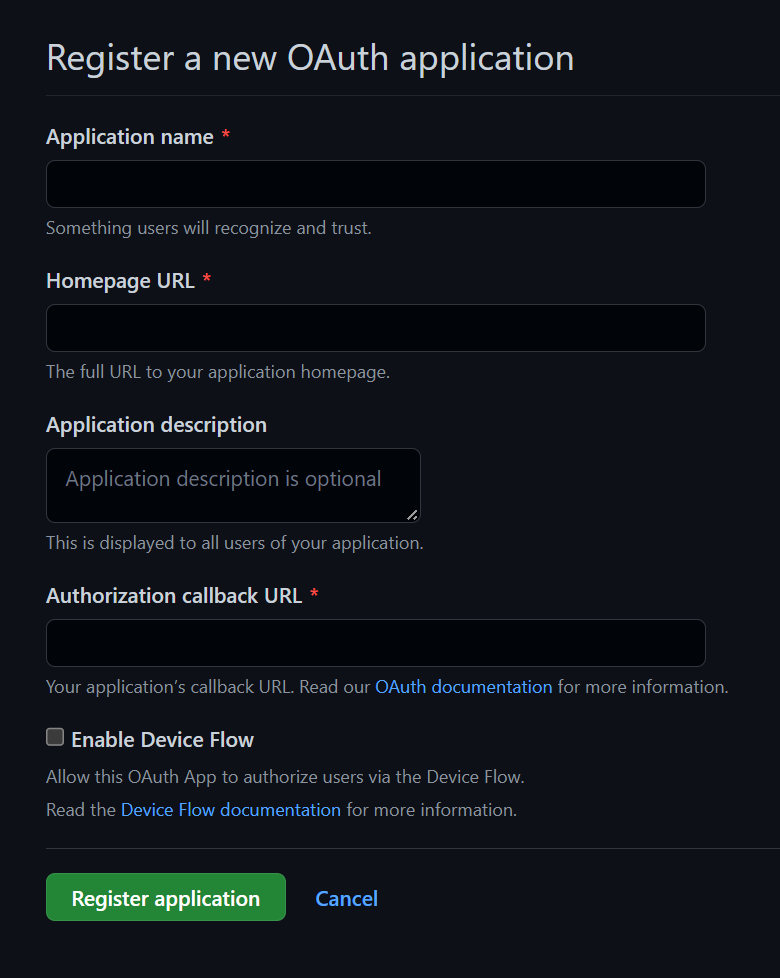
* For **Authorization callback URL**:
* For development, put: `http://localhost:3001/auth/github/callback`.
* Once you know on which URL your API server will be deployed, you can create a new app with that URL instead e.g. `https://your-server-url.com/auth/github/callback`.
4. Hit **Register application**.
5. Hit **Generate a new client secret** on the next page.
6. Copy your Client ID and Client secret as you'll need them in the next step.
### 4\. Adding Environment Variables[](#4-adding-environment-variables "Direct link to 4. Adding Environment Variables")
Add these environment variables to the `.env.server` file at the root of your project (take their values from the previous step):
.env.server
```
GITHUB_CLIENT_ID=your-github-client-idGITHUB_CLIENT_SECRET=your-github-client-secret
```
### 5\. Adding the Necessary Routes and Pages[](#5-adding-the-necessary-routes-and-pages "Direct link to 5. Adding the Necessary Routes and Pages")
Let's define the necessary authentication Routes and Pages.
Add the following code to your `main.wasp` file:
* JavaScript
* TypeScript
main.wasp
```
// ...// 6. Define the routesroute LoginRoute { path: "/login", to: LoginPage }page LoginPage { component: import { Login } from "@src/pages/auth.jsx"}
```
We'll define the React components for these pages in the `src/pages/auth.tsx` file below.
### 6\. Creating the Client Pages[](#6-creating-the-client-pages "Direct link to 6. Creating the Client Pages")
info
We are using [Tailwind CSS](https://tailwindcss.com/) to style the pages. Read more about how to add it [here](https://wasp-lang.dev/docs/project/css-frameworks).
Let's create a `auth.tsx` file in the `src/pages` folder and add the following to it:
* JavaScript
* TypeScript
src/pages/auth.jsx
```
import { LoginForm } from 'wasp/client/auth'export function Login() { return ( <Layout> <LoginForm /> </Layout> )}// A layout component to center the contentexport function Layout({ children }) { return ( <div className="w-full h-full bg-white"> <div className="min-w-full min-h-[75vh] flex items-center justify-center"> <div className="w-full h-full max-w-sm p-5 bg-white"> <div>{children}</div> </div> </div> </div> )}
```
We imported the generated Auth UI components and used them in our pages. Read more about the Auth UI components [here](https://wasp-lang.dev/docs/auth/ui).
### Conclusion[](#conclusion "Direct link to Conclusion")
Yay, we've successfully set up Github Auth! 🎉
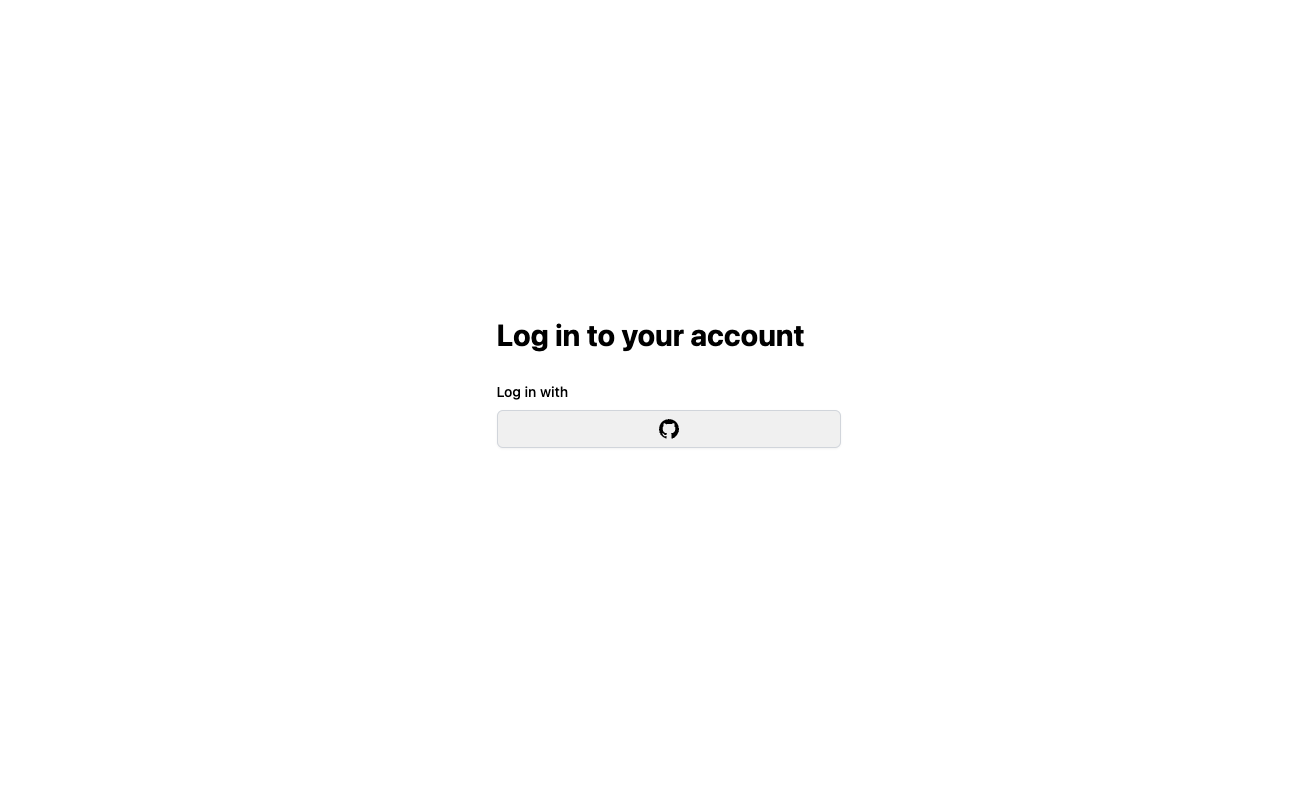
Running `wasp db migrate-dev` and `wasp start` should now give you a working app with authentication. To see how to protect specific pages (i.e., hide them from non-authenticated users), read the docs on [using auth](https://wasp-lang.dev/docs/auth/overview).
## Default Behaviour[](#default-behaviour "Direct link to Default Behaviour")
Add `gitHub: {}` to the `auth.methods` dictionary to use it with default settings.
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { gitHub: {} }, onAuthFailedRedirectTo: "/login" },}
```
When a user **signs in for the first time**, Wasp creates a new user account and links it to the chosen auth provider account for future logins.
## Overrides[](#overrides "Direct link to Overrides")
By default, Wasp doesn't store any information it receives from the social login provider. It only stores the user's ID specific to the provider.
There are two mechanisms used for overriding the default behavior:
* `userSignupFields`
* `configFn`
Let's explore them in more detail.
### Data Received From GitHub[](#data-received-from-github "Direct link to Data Received From GitHub")
We are using GitHub's API and its `/user` and `/user/emails` endpoints to get the user data.
We combine the data from the two endpoints
You'll find the emails in the `emails` property in the object that you receive in `userSignupFields`.
This is because we combine the data from the `/user` and `/user/emails` endpoints **if the `user` or `user:email` scope is requested.**
The data we receive from GitHub on the `/user` endpoint looks something this:
```
{ "login": "octocat", "id": 1, "name": "monalisa octocat", "avatar_url": "https://github.com/images/error/octocat_happy.gif", "gravatar_id": "", // ...}
```
And the data from the `/user/emails` endpoint looks something like this:
```
[ { "email": "octocat@github.com", "verified": true, "primary": true, "visibility": "public" }]
```
The fields you receive will depend on the scopes you requested. By default we don't specify any scopes. If you want to get the emails, you need to specify the `user` or `user:email` scope in the `configFn` function.
For an up to date info about the data received from GitHub, please refer to the [GitHub API documentation](https://docs.github.com/en/rest/users/users?apiVersion=2022-11-28#get-the-authenticated-user).
### Using the Data Received From GitHub[](#using-the-data-received-from-github "Direct link to Using the Data Received From GitHub")
When a user logs in using a social login provider, the backend receives some data about the user. Wasp lets you access this data inside the `userSignupFields` getters.
For example, the User entity can include a `displayName` field which you can set based on the details received from the provider.
Wasp also lets you customize the configuration of the providers' settings using the `configFn` function.
Let's use this example to show both fields in action:
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { gitHub: { configFn: import { getConfig } from "@src/auth/github.js", userSignupFields: import { userSignupFields } from "@src/auth/github.js" } }, onAuthFailedRedirectTo: "/login" },}entity User {=psl id Int @id @default(autoincrement()) username String @unique displayName Stringpsl=}// ...
```
src/auth/github.js
```
export const userSignupFields = { username: () => "hardcoded-username", displayName: (data) => data.profile.name,};export function getConfig() { return { scopes: ['user'], };}
```
## Using Auth[](#using-auth "Direct link to Using Auth")
To read more about how to set up the logout button and get access to the logged-in user in both client and server code, read the docs on [using auth](https://wasp-lang.dev/docs/auth/overview).
## API Reference[](#api-reference "Direct link to API Reference")
Provider-specific behavior comes down to implementing two functions.
* `configFn`
* `userSignupFields`
The reference shows how to define both.
For behavior common to all providers, check the general [API Reference](https://wasp-lang.dev/docs/auth/overview#api-reference).
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { gitHub: { configFn: import { getConfig } from "@src/auth/github.js", userSignupFields: import { userSignupFields } from "@src/auth/github.js" } }, onAuthFailedRedirectTo: "/login" },}
```
The `gitHub` dict has the following properties:
* #### `configFn: ExtImport`[](#configfn-extimport "Direct link to configfn-extimport")
This function should return an object with the scopes for the OAuth provider.
* JavaScript
* TypeScript
src/auth/github.js
```
export function getConfig() { return { scopes: [], }}
```
* #### `userSignupFields: ExtImport`[](#usersignupfields-extimport "Direct link to usersignupfields-extimport")
`userSignupFields` defines all the extra fields that need to be set on the `User` during the sign-up process. For example, if you have `address` and `phone` fields on your `User` entity, you can set them by defining the `userSignupFields` like this:
* JavaScript
* TypeScript
src/auth.js
```
import { defineUserSignupFields } from 'wasp/server/auth'export const userSignupFields = defineUserSignupFields({ address: (data) => { if (!data.address) { throw new Error('Address is required') } return data.address } phone: (data) => data.phone,})
```
Read more about the `userSignupFields` function [here](https://wasp-lang.dev/docs/auth/overview#1-defining-extra-fields). | null | https://wasp-lang.dev/docs/auth/social-auth/github | Wasp supports Github Authentication out of the box. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecd2acc354bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:07 GMT | Tue, 23 Apr 2024 15:24:07 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=ySefw1Ll4oOAiPtV%2F70WtDTSmZqQw3zFeGPF%2Bl5bZsDF4avaTP3QRPCDlFDEA3QByDVR9dSWzepjT6hICflMpUUXhmOQ%2Fw%2BOmjcYj1vwv2hw5Kdo3mu8%2B6kgOrmlsE4R"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | 9593c6e72232cd2457529c5a06d562d384a1b0b8 | h2 | CAF8:83772:3990B8A:44AD815:6627D03E | HIT | MISS | cache-nyc-kteb1890087-NYC | S1713885247.182391, VS0, VE18 | null | null | en | og:image | https://wasp-lang.dev/docs/auth/social-auth/github | og:url | GitHub | Wasp | og:title | Wasp supports Github Authentication out of the box. | og:description | GitHub | Wasp | null | GitHub
Wasp supports Github Authentication out of the box. GitHub is a great external auth choice when you're building apps for developers, as most of them already have a GitHub account.
Letting your users log in using their GitHub accounts turns the signup process into a breeze.
Let's walk through enabling Github Authentication, explain some of the default settings, and show how to override them.
Setting up Github Auth
Enabling GitHub Authentication comes down to a series of steps:
Enabling GitHub authentication in the Wasp file.
Adding the User entity.
Creating a GitHub OAuth app.
Adding the necessary Routes and Pages
Using Auth UI components in our Pages.
Here's a skeleton of how our main.wasp should look like after we're done:
main.wasp
// Configuring the social authentication
app myApp {
auth: { ... }
}
// Defining entities
entity User { ... }
// Defining routes and pages
route LoginRoute { ... }
page LoginPage { ... }
1. Adding Github Auth to Your Wasp File
Let's start by properly configuring the Auth object:
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
// 1. Specify the User entity (we'll define it next)
userEntity: User,
methods: {
// 2. Enable Github Auth
gitHub: {}
},
onAuthFailedRedirectTo: "/login"
},
}
2. Add the User Entity
Let's now define the app.auth.userEntity entity:
JavaScript
TypeScript
main.wasp
// ...
// 3. Define the User entity
entity User {=psl
id Int @id @default(autoincrement())
// ...
psl=}
3. Creating a GitHub OAuth App
To use GitHub as an authentication method, you'll first need to create a GitHub OAuth App and provide Wasp with your client key and secret. Here's how you do it:
Log into your GitHub account and navigate to: https://github.com/settings/developers.
Select New OAuth App.
Supply required information.
For Authorization callback URL:
For development, put: http://localhost:3001/auth/github/callback.
Once you know on which URL your API server will be deployed, you can create a new app with that URL instead e.g. https://your-server-url.com/auth/github/callback.
Hit Register application.
Hit Generate a new client secret on the next page.
Copy your Client ID and Client secret as you'll need them in the next step.
4. Adding Environment Variables
Add these environment variables to the .env.server file at the root of your project (take their values from the previous step):
.env.server
GITHUB_CLIENT_ID=your-github-client-id
GITHUB_CLIENT_SECRET=your-github-client-secret
5. Adding the Necessary Routes and Pages
Let's define the necessary authentication Routes and Pages.
Add the following code to your main.wasp file:
JavaScript
TypeScript
main.wasp
// ...
// 6. Define the routes
route LoginRoute { path: "/login", to: LoginPage }
page LoginPage {
component: import { Login } from "@src/pages/auth.jsx"
}
We'll define the React components for these pages in the src/pages/auth.tsx file below.
6. Creating the Client Pages
info
We are using Tailwind CSS to style the pages. Read more about how to add it here.
Let's create a auth.tsx file in the src/pages folder and add the following to it:
JavaScript
TypeScript
src/pages/auth.jsx
import { LoginForm } from 'wasp/client/auth'
export function Login() {
return (
<Layout>
<LoginForm />
</Layout>
)
}
// A layout component to center the content
export function Layout({ children }) {
return (
<div className="w-full h-full bg-white">
<div className="min-w-full min-h-[75vh] flex items-center justify-center">
<div className="w-full h-full max-w-sm p-5 bg-white">
<div>{children}</div>
</div>
</div>
</div>
)
}
We imported the generated Auth UI components and used them in our pages. Read more about the Auth UI components here.
Conclusion
Yay, we've successfully set up Github Auth! 🎉
Running wasp db migrate-dev and wasp start should now give you a working app with authentication. To see how to protect specific pages (i.e., hide them from non-authenticated users), read the docs on using auth.
Default Behaviour
Add gitHub: {} to the auth.methods dictionary to use it with default settings.
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
gitHub: {}
},
onAuthFailedRedirectTo: "/login"
},
}
When a user signs in for the first time, Wasp creates a new user account and links it to the chosen auth provider account for future logins.
Overrides
By default, Wasp doesn't store any information it receives from the social login provider. It only stores the user's ID specific to the provider.
There are two mechanisms used for overriding the default behavior:
userSignupFields
configFn
Let's explore them in more detail.
Data Received From GitHub
We are using GitHub's API and its /user and /user/emails endpoints to get the user data.
We combine the data from the two endpoints
You'll find the emails in the emails property in the object that you receive in userSignupFields.
This is because we combine the data from the /user and /user/emails endpoints if the user or user:email scope is requested.
The data we receive from GitHub on the /user endpoint looks something this:
{
"login": "octocat",
"id": 1,
"name": "monalisa octocat",
"avatar_url": "https://github.com/images/error/octocat_happy.gif",
"gravatar_id": "",
// ...
}
And the data from the /user/emails endpoint looks something like this:
[
{
"email": "octocat@github.com",
"verified": true,
"primary": true,
"visibility": "public"
}
]
The fields you receive will depend on the scopes you requested. By default we don't specify any scopes. If you want to get the emails, you need to specify the user or user:email scope in the configFn function.
For an up to date info about the data received from GitHub, please refer to the GitHub API documentation.
Using the Data Received From GitHub
When a user logs in using a social login provider, the backend receives some data about the user. Wasp lets you access this data inside the userSignupFields getters.
For example, the User entity can include a displayName field which you can set based on the details received from the provider.
Wasp also lets you customize the configuration of the providers' settings using the configFn function.
Let's use this example to show both fields in action:
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
gitHub: {
configFn: import { getConfig } from "@src/auth/github.js",
userSignupFields: import { userSignupFields } from "@src/auth/github.js"
}
},
onAuthFailedRedirectTo: "/login"
},
}
entity User {=psl
id Int @id @default(autoincrement())
username String @unique
displayName String
psl=}
// ...
src/auth/github.js
export const userSignupFields = {
username: () => "hardcoded-username",
displayName: (data) => data.profile.name,
};
export function getConfig() {
return {
scopes: ['user'],
};
}
Using Auth
To read more about how to set up the logout button and get access to the logged-in user in both client and server code, read the docs on using auth.
API Reference
Provider-specific behavior comes down to implementing two functions.
configFn
userSignupFields
The reference shows how to define both.
For behavior common to all providers, check the general API Reference.
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
gitHub: {
configFn: import { getConfig } from "@src/auth/github.js",
userSignupFields: import { userSignupFields } from "@src/auth/github.js"
}
},
onAuthFailedRedirectTo: "/login"
},
}
The gitHub dict has the following properties:
configFn: ExtImport
This function should return an object with the scopes for the OAuth provider.
JavaScript
TypeScript
src/auth/github.js
export function getConfig() {
return {
scopes: [],
}
}
userSignupFields: ExtImport
userSignupFields defines all the extra fields that need to be set on the User during the sign-up process. For example, if you have address and phone fields on your User entity, you can set them by defining the userSignupFields like this:
JavaScript
TypeScript
src/auth.js
import { defineUserSignupFields } from 'wasp/server/auth'
export const userSignupFields = defineUserSignupFields({
address: (data) => {
if (!data.address) {
throw new Error('Address is required')
}
return data.address
}
phone: (data) => data.phone,
})
Read more about the userSignupFields function here. | https://wasp-lang.dev/docs/auth/social-auth/github |
|
1 | null | 2024-04-23T15:14:18.123Z | https://wasp-lang.dev/docs/auth/social-auth/keycloak | https://wasp-lang.dev/docs | http | ## Keycloak
Wasp supports Keycloak Authentication out of the box.
[Keycloak](https://www.keycloak.org/) is an open-source identity and access management solution for modern applications and services. Keycloak provides both SAML and OpenID protocol solutions. It also has a very flexible and powerful administration UI.
Let's walk through enabling Keycloak authentication, explain some of the default settings, and show how to override them.
## Setting up Keycloak Auth[](#setting-up-keycloak-auth "Direct link to Setting up Keycloak Auth")
Enabling Keycloak Authentication comes down to a series of steps:
1. Enabling Keycloak authentication in the Wasp file.
2. Adding the `User` entity.
3. Creating a Keycloak client.
4. Adding the necessary Routes and Pages
5. Using Auth UI components in our Pages.
Here's a skeleton of how our `main.wasp` should look like after we're done:
main.wasp
```
// Configuring the social authenticationapp myApp { auth: { ... }}// Defining entitiesentity User { ... }// Defining routes and pagesroute LoginRoute { ... }page LoginPage { ... }
```
### 1\. Adding Keycloak Auth to Your Wasp File[](#1-adding-keycloak-auth-to-your-wasp-file "Direct link to 1. Adding Keycloak Auth to Your Wasp File")
Let's start by properly configuring the Auth object:
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { // 1. Specify the User entity (we'll define it next) userEntity: User, methods: { // 2. Enable Keycloak Auth keycloak: {} }, onAuthFailedRedirectTo: "/login" },}
```
The `userEntity` is explained in [the social auth overview](https://wasp-lang.dev/docs/auth/social-auth/overview#social-login-entity).
### 2\. Adding the User Entity[](#2-adding-the-user-entity "Direct link to 2. Adding the User Entity")
Let's now define the `app.auth.userEntity` entity:
* JavaScript
* TypeScript
main.wasp
```
// ...// 3. Define the User entityentity User {=psl id Int @id @default(autoincrement()) // ...psl=}
```
### 3\. Creating a Keycloak Client[](#3-creating-a-keycloak-client "Direct link to 3. Creating a Keycloak Client")
1. Log into your Keycloak admin console.
2. Under **Clients**, click on **Create Client**.
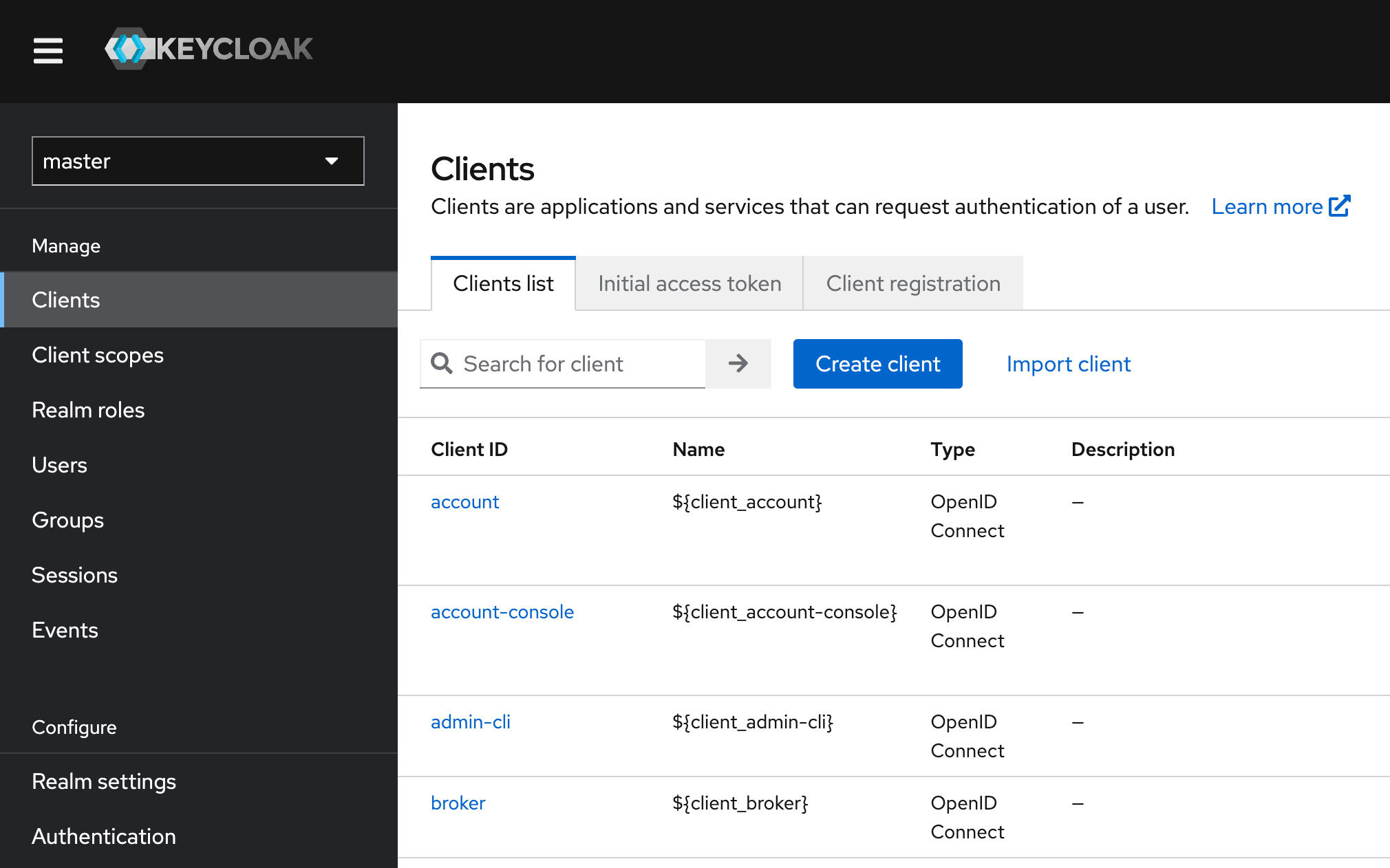
3. Fill in the **Client ID** and choose a name for the client.
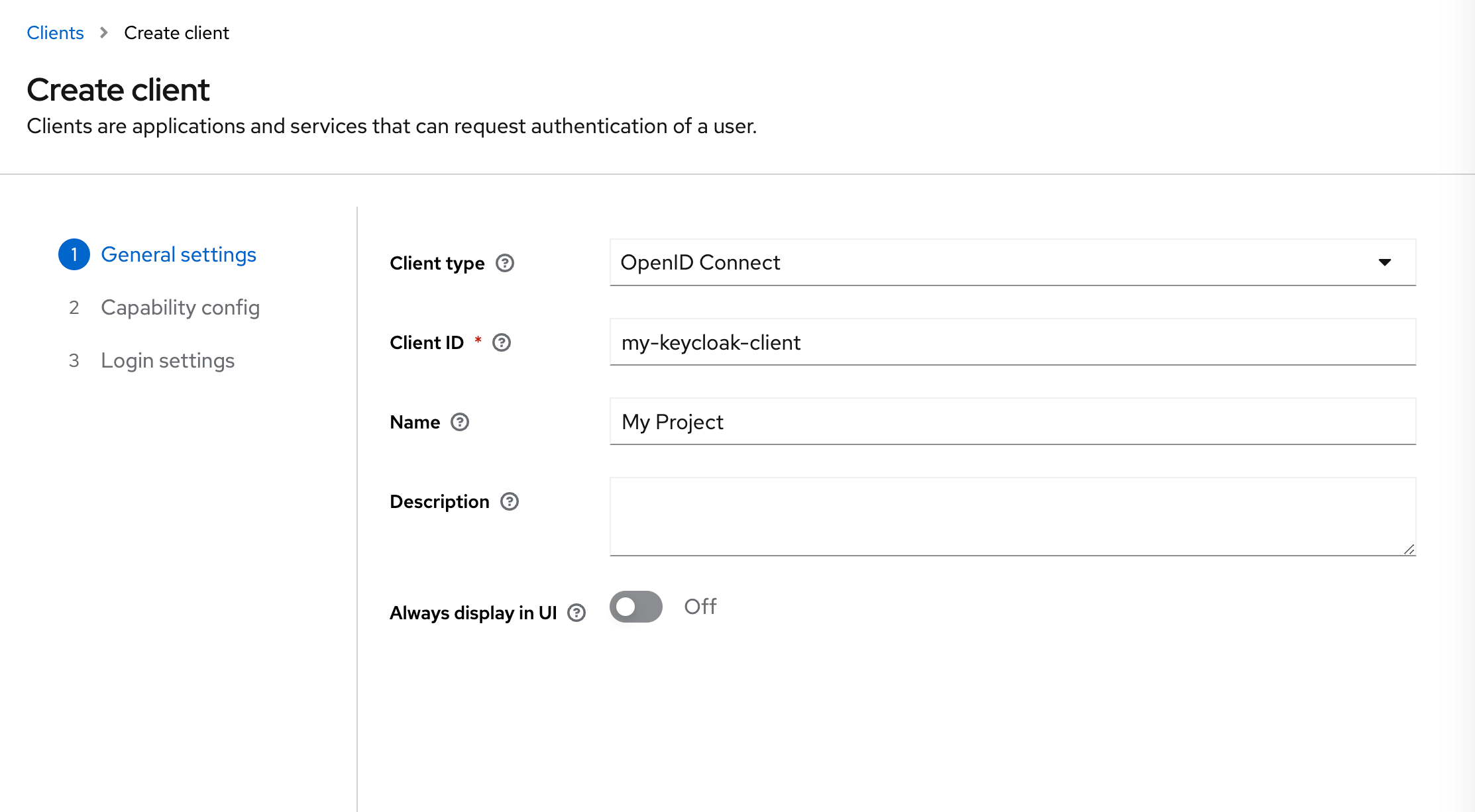
4. In the next step, enable **Client Authentication**.
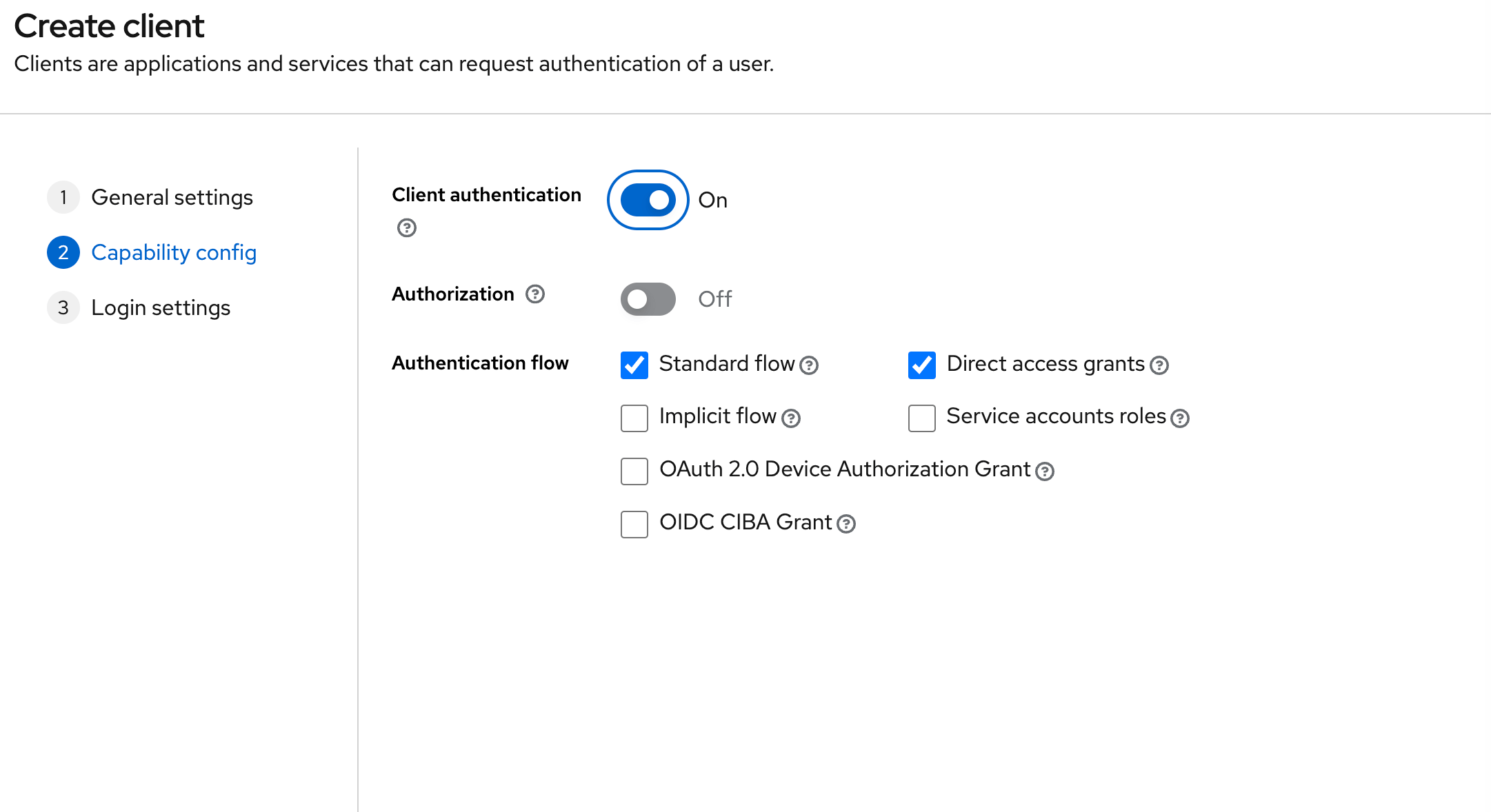
5. Under **Valid Redirect URIs**, add `http://localhost:3001/auth/keycloak/callback` for local development.
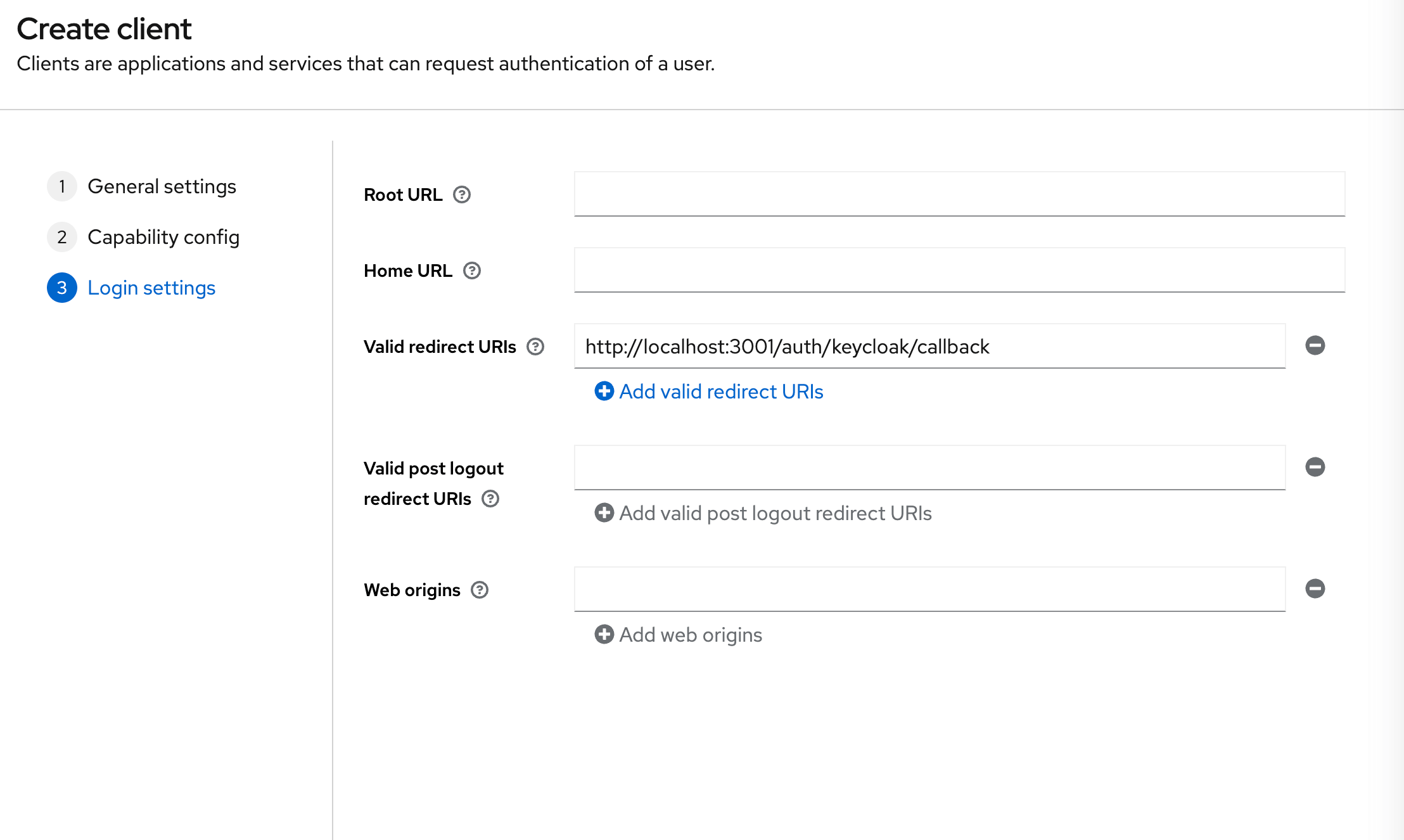
* Once you know on which URL(s) your API server will be deployed, also add those URL(s).
* For example: `https://my-server-url.com/auth/keycloak/callback`.
6. Click **Save**.
7. In the **Credentials** tab, copy the **Client Secret** value, which we'll use in the next step.
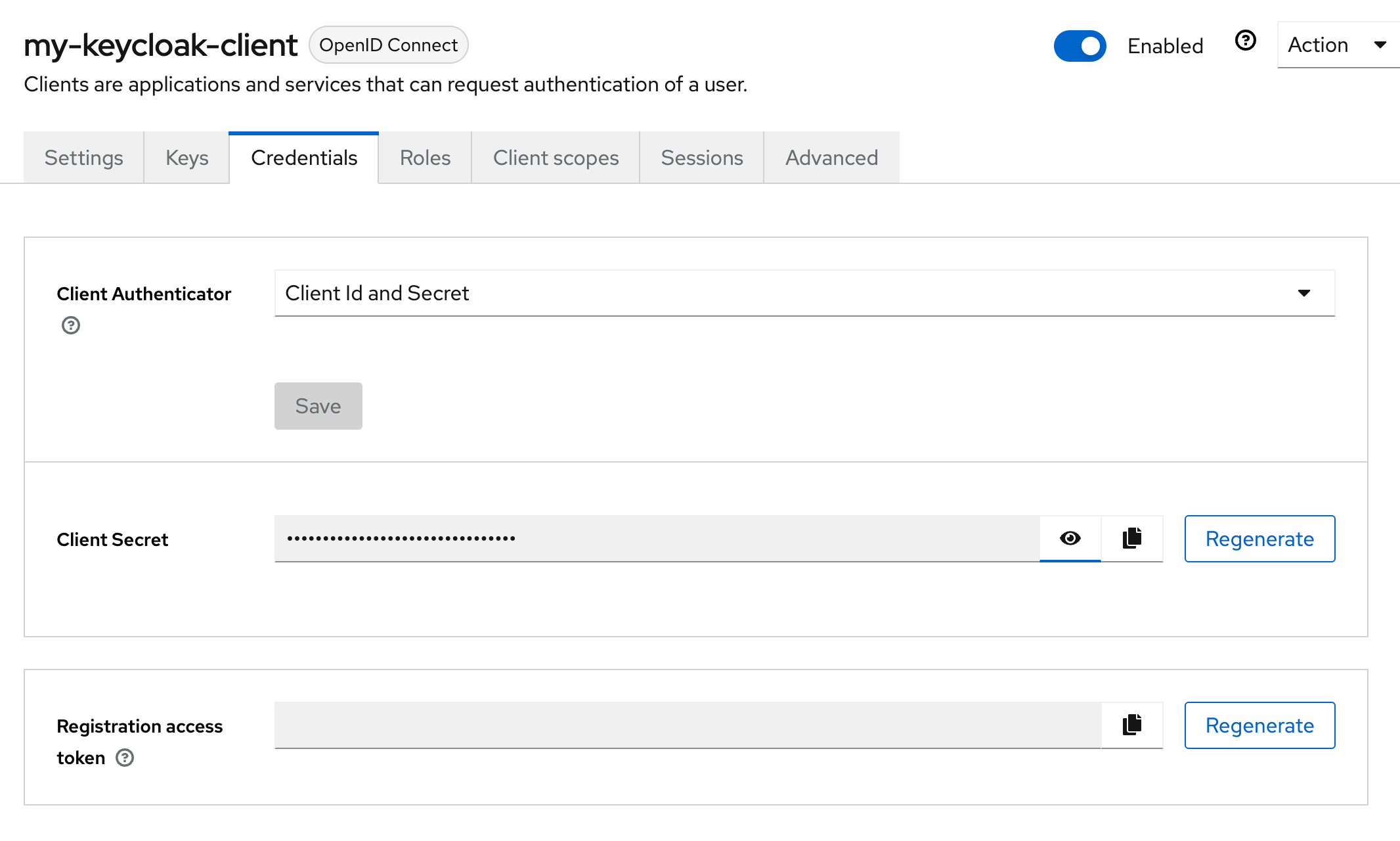
### 4\. Adding Environment Variables[](#4-adding-environment-variables "Direct link to 4. Adding Environment Variables")
Add these environment variables to the `.env.server` file at the root of your project (take their values from the previous step):
.env.server
```
KEYCLOAK_CLIENT_ID=your-keycloak-client-idKEYCLOAK_CLIENT_SECRET=your-keycloak-client-secretKEYCLOAK_REALM_URL=https://your-keycloak-url.com/realms/master
```
We assumed in the `KEYCLOAK_REALM_URL` env variable that you are using the `master` realm. If you are using a different realm, replace `master` with your realm name.
### 5\. Adding the Necessary Routes and Pages[](#5-adding-the-necessary-routes-and-pages "Direct link to 5. Adding the Necessary Routes and Pages")
Let's define the necessary authentication Routes and Pages.
Add the following code to your `main.wasp` file:
* JavaScript
* TypeScript
main.wasp
```
// ...// 6. Define the routesroute LoginRoute { path: "/login", to: LoginPage }page LoginPage { component: import { Login } from "@src/pages/auth.jsx"}
```
We'll define the React components for these pages in the `src/pages/auth.tsx` file below.
### 6\. Create the Client Pages[](#6-create-the-client-pages "Direct link to 6. Create the Client Pages")
info
We are using [Tailwind CSS](https://tailwindcss.com/) to style the pages. Read more about how to add it [here](https://wasp-lang.dev/docs/project/css-frameworks).
Let's now create an `auth.tsx` file in the `src/pages`. It should have the following code:
* JavaScript
* TypeScript
src/pages/auth.jsx
```
import { LoginForm } from 'wasp/client/auth'export function Login() { return ( <Layout> <LoginForm /> </Layout> )}// A layout component to center the contentexport function Layout({ children }) { return ( <div className="w-full h-full bg-white"> <div className="min-w-full min-h-[75vh] flex items-center justify-center"> <div className="w-full h-full max-w-sm p-5 bg-white"> <div>{children}</div> </div> </div> </div> )}
```
Auth UI
Our pages use an automatically generated Auth UI component. Read more about Auth UI components [here](https://wasp-lang.dev/docs/auth/ui).
### Conclusion[](#conclusion "Direct link to Conclusion")
Yay, we've successfully set up Keycloak Auth!
Running `wasp db migrate-dev` and `wasp start` should now give you a working app with authentication. To see how to protect specific pages (i.e., hide them from non-authenticated users), read the docs on [using auth](https://wasp-lang.dev/docs/auth/overview).
## Default Behaviour[](#default-behaviour "Direct link to Default Behaviour")
Add `keycloak: {}` to the `auth.methods` dictionary to use it with default settings:
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { keycloak: {} }, onAuthFailedRedirectTo: "/login" },}
```
When a user **signs in for the first time**, Wasp creates a new user account and links it to the chosen auth provider account for future logins.
## Overrides[](#overrides "Direct link to Overrides")
By default, Wasp doesn't store any information it receives from the social login provider. It only stores the user's ID specific to the provider.
There are two mechanisms used for overriding the default behavior:
* `userSignupFields`
* `configFn`
Let's explore them in more detail.
### Data Received From Keycloak[](#data-received-from-keycloak "Direct link to Data Received From Keycloak")
We are using Keycloak's API and its `/userinfo` endpoint to fetch the user's data.
Keycloak user data
```
{ sub: '5adba8fc-3ea6-445a-a379-13f0bb0b6969', email_verified: true, name: 'Test User', preferred_username: 'test', given_name: 'Test', family_name: 'User', email: '[email protected]'}
```
The fields you receive will depend on the scopes you requested. The default scope is set to `profile` only. If you want to get the user's email, you need to specify the `email` scope in the `configFn` function.
For up-to-date info about the data received from Keycloak, please refer to the [Keycloak API documentation](https://www.keycloak.org/docs-api/23.0.7/javadocs/org/keycloak/representations/UserInfo.html).
### Using the Data Received From Keycloak[](#using-the-data-received-from-keycloak "Direct link to Using the Data Received From Keycloak")
When a user logs in using a social login provider, the backend receives some data about the user. Wasp lets you access this data inside the `userSignupFields` getters.
For example, the User entity can include a `displayName` field which you can set based on the details received from the provider.
Wasp also lets you customize the configuration of the providers' settings using the `configFn` function.
Let's use this example to show both fields in action:
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { keycloak: { configFn: import { getConfig } from "@src/auth/keycloak.js", userSignupFields: import { userSignupFields } from "@src/auth/keycloak.js" } }, onAuthFailedRedirectTo: "/login" },}entity User {=psl id Int @id @default(autoincrement()) username String @unique displayName Stringpsl=}// ...
```
src/auth/keycloak.js
```
export const userSignupFields = { username: () => "hardcoded-username", displayName: (data) => data.profile.name,}export function getConfig() { return { scopes: ['profile', 'email'], }}
```
## Using Auth[](#using-auth "Direct link to Using Auth")
To read more about how to set up the logout button and get access to the logged-in user in both client and server code, read the docs on [using auth](https://wasp-lang.dev/docs/auth/overview).
## API Reference[](#api-reference "Direct link to API Reference")
Provider-specific behavior comes down to implementing two functions.
* `configFn`
* `userSignupFields`
The reference shows how to define both.
For behavior common to all providers, check the general [API Reference](https://wasp-lang.dev/docs/auth/overview#api-reference).
* JavaScript
* TypeScript
main.wasp
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", auth: { userEntity: User, methods: { keycloak: { configFn: import { getConfig } from "@src/auth/keycloak.js", userSignupFields: import { userSignupFields } from "@src/auth/keycloak.js" } }, onAuthFailedRedirectTo: "/login" },}
```
The `keycloak` dict has the following properties:
* #### `configFn: ExtImport`[](#configfn-extimport "Direct link to configfn-extimport")
This function must return an object with the scopes for the OAuth provider.
* JavaScript
* TypeScript
src/auth/keycloak.js
```
export function getConfig() { return { scopes: ['profile', 'email'], }}
```
* #### `userSignupFields: ExtImport`[](#usersignupfields-extimport "Direct link to usersignupfields-extimport")
`userSignupFields` defines all the extra fields that need to be set on the `User` during the sign-up process. For example, if you have `address` and `phone` fields on your `User` entity, you can set them by defining the `userSignupFields` like this:
* JavaScript
* TypeScript
src/auth.js
```
import { defineUserSignupFields } from 'wasp/server/auth'export const userSignupFields = defineUserSignupFields({ address: (data) => { if (!data.address) { throw new Error('Address is required') } return data.address } phone: (data) => data.phone,})
```
Read more about the `userSignupFields` function [here](https://wasp-lang.dev/docs/auth/overview#1-defining-extra-fields). | null | https://wasp-lang.dev/docs/auth/social-auth/keycloak | Wasp supports Keycloak Authentication out of the box. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecd6ecbb756d4-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:18 GMT | Tue, 23 Apr 2024 15:17:26 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=NjLilRkViXBMCbKr5KH%2Ba5BuJvmbt5iVR0jPNm6AcSJNAfprPoqED3ukMJRaw%2FtnWBZzheBo%2FqyBXerzJd2Qtaq8nlZrQBolVYGdUP7HyFZqHhzqVSIbcmxxqJz7DlbT"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | HIT | 0 | de5c85e90a6151a3e20d0496c079b3c2ed53f494 | null | 3D8E:16EE:844396:9E9DE6:6627CEAC | null | MISS | cache-iad-kiad7000173-IAD | S1713885258.064817,VS0,VE6 | null | null | en | og:image | https://wasp-lang.dev/docs/auth/social-auth/keycloak | og:url | Keycloak | Wasp | og:title | Wasp supports Keycloak Authentication out of the box. | og:description | Keycloak | Wasp | null | Keycloak
Wasp supports Keycloak Authentication out of the box.
Keycloak is an open-source identity and access management solution for modern applications and services. Keycloak provides both SAML and OpenID protocol solutions. It also has a very flexible and powerful administration UI.
Let's walk through enabling Keycloak authentication, explain some of the default settings, and show how to override them.
Setting up Keycloak Auth
Enabling Keycloak Authentication comes down to a series of steps:
Enabling Keycloak authentication in the Wasp file.
Adding the User entity.
Creating a Keycloak client.
Adding the necessary Routes and Pages
Using Auth UI components in our Pages.
Here's a skeleton of how our main.wasp should look like after we're done:
main.wasp
// Configuring the social authentication
app myApp {
auth: { ... }
}
// Defining entities
entity User { ... }
// Defining routes and pages
route LoginRoute { ... }
page LoginPage { ... }
1. Adding Keycloak Auth to Your Wasp File
Let's start by properly configuring the Auth object:
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
// 1. Specify the User entity (we'll define it next)
userEntity: User,
methods: {
// 2. Enable Keycloak Auth
keycloak: {}
},
onAuthFailedRedirectTo: "/login"
},
}
The userEntity is explained in the social auth overview.
2. Adding the User Entity
Let's now define the app.auth.userEntity entity:
JavaScript
TypeScript
main.wasp
// ...
// 3. Define the User entity
entity User {=psl
id Int @id @default(autoincrement())
// ...
psl=}
3. Creating a Keycloak Client
Log into your Keycloak admin console.
Under Clients, click on Create Client.
Fill in the Client ID and choose a name for the client.
In the next step, enable Client Authentication.
Under Valid Redirect URIs, add http://localhost:3001/auth/keycloak/callback for local development.
Once you know on which URL(s) your API server will be deployed, also add those URL(s).
For example: https://my-server-url.com/auth/keycloak/callback.
Click Save.
In the Credentials tab, copy the Client Secret value, which we'll use in the next step.
4. Adding Environment Variables
Add these environment variables to the .env.server file at the root of your project (take their values from the previous step):
.env.server
KEYCLOAK_CLIENT_ID=your-keycloak-client-id
KEYCLOAK_CLIENT_SECRET=your-keycloak-client-secret
KEYCLOAK_REALM_URL=https://your-keycloak-url.com/realms/master
We assumed in the KEYCLOAK_REALM_URL env variable that you are using the master realm. If you are using a different realm, replace master with your realm name.
5. Adding the Necessary Routes and Pages
Let's define the necessary authentication Routes and Pages.
Add the following code to your main.wasp file:
JavaScript
TypeScript
main.wasp
// ...
// 6. Define the routes
route LoginRoute { path: "/login", to: LoginPage }
page LoginPage {
component: import { Login } from "@src/pages/auth.jsx"
}
We'll define the React components for these pages in the src/pages/auth.tsx file below.
6. Create the Client Pages
info
We are using Tailwind CSS to style the pages. Read more about how to add it here.
Let's now create an auth.tsx file in the src/pages. It should have the following code:
JavaScript
TypeScript
src/pages/auth.jsx
import { LoginForm } from 'wasp/client/auth'
export function Login() {
return (
<Layout>
<LoginForm />
</Layout>
)
}
// A layout component to center the content
export function Layout({ children }) {
return (
<div className="w-full h-full bg-white">
<div className="min-w-full min-h-[75vh] flex items-center justify-center">
<div className="w-full h-full max-w-sm p-5 bg-white">
<div>{children}</div>
</div>
</div>
</div>
)
}
Auth UI
Our pages use an automatically generated Auth UI component. Read more about Auth UI components here.
Conclusion
Yay, we've successfully set up Keycloak Auth!
Running wasp db migrate-dev and wasp start should now give you a working app with authentication. To see how to protect specific pages (i.e., hide them from non-authenticated users), read the docs on using auth.
Default Behaviour
Add keycloak: {} to the auth.methods dictionary to use it with default settings:
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
keycloak: {}
},
onAuthFailedRedirectTo: "/login"
},
}
When a user signs in for the first time, Wasp creates a new user account and links it to the chosen auth provider account for future logins.
Overrides
By default, Wasp doesn't store any information it receives from the social login provider. It only stores the user's ID specific to the provider.
There are two mechanisms used for overriding the default behavior:
userSignupFields
configFn
Let's explore them in more detail.
Data Received From Keycloak
We are using Keycloak's API and its /userinfo endpoint to fetch the user's data.
Keycloak user data
{
sub: '5adba8fc-3ea6-445a-a379-13f0bb0b6969',
email_verified: true,
name: 'Test User',
preferred_username: 'test',
given_name: 'Test',
family_name: 'User',
email: '[email protected]'
}
The fields you receive will depend on the scopes you requested. The default scope is set to profile only. If you want to get the user's email, you need to specify the email scope in the configFn function.
For up-to-date info about the data received from Keycloak, please refer to the Keycloak API documentation.
Using the Data Received From Keycloak
When a user logs in using a social login provider, the backend receives some data about the user. Wasp lets you access this data inside the userSignupFields getters.
For example, the User entity can include a displayName field which you can set based on the details received from the provider.
Wasp also lets you customize the configuration of the providers' settings using the configFn function.
Let's use this example to show both fields in action:
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
keycloak: {
configFn: import { getConfig } from "@src/auth/keycloak.js",
userSignupFields: import { userSignupFields } from "@src/auth/keycloak.js"
}
},
onAuthFailedRedirectTo: "/login"
},
}
entity User {=psl
id Int @id @default(autoincrement())
username String @unique
displayName String
psl=}
// ...
src/auth/keycloak.js
export const userSignupFields = {
username: () => "hardcoded-username",
displayName: (data) => data.profile.name,
}
export function getConfig() {
return {
scopes: ['profile', 'email'],
}
}
Using Auth
To read more about how to set up the logout button and get access to the logged-in user in both client and server code, read the docs on using auth.
API Reference
Provider-specific behavior comes down to implementing two functions.
configFn
userSignupFields
The reference shows how to define both.
For behavior common to all providers, check the general API Reference.
JavaScript
TypeScript
main.wasp
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
keycloak: {
configFn: import { getConfig } from "@src/auth/keycloak.js",
userSignupFields: import { userSignupFields } from "@src/auth/keycloak.js"
}
},
onAuthFailedRedirectTo: "/login"
},
}
The keycloak dict has the following properties:
configFn: ExtImport
This function must return an object with the scopes for the OAuth provider.
JavaScript
TypeScript
src/auth/keycloak.js
export function getConfig() {
return {
scopes: ['profile', 'email'],
}
}
userSignupFields: ExtImport
userSignupFields defines all the extra fields that need to be set on the User during the sign-up process. For example, if you have address and phone fields on your User entity, you can set them by defining the userSignupFields like this:
JavaScript
TypeScript
src/auth.js
import { defineUserSignupFields } from 'wasp/server/auth'
export const userSignupFields = defineUserSignupFields({
address: (data) => {
if (!data.address) {
throw new Error('Address is required')
}
return data.address
}
phone: (data) => data.phone,
})
Read more about the userSignupFields function here. | https://wasp-lang.dev/docs/auth/social-auth/keycloak |
|
1 | null | 2024-04-23T15:14:20.532Z | https://wasp-lang.dev/docs/auth/entities | https://wasp-lang.dev/docs | http | ## Auth Entities
Wasp supports multiple different authentication methods and for each method, we need to store different information about the user. For example, if you are using the [Username & password](https://wasp-lang.dev/docs/auth/username-and-pass) authentication method, we need to store the user's username and password. On the other hand, if you are using the [Email](https://wasp-lang.dev/docs/auth/email) authentication method, you will need to store the user's email, password and for example, their email verification status.
## Entities Explained[](#entities-explained "Direct link to Entities Explained")
To store user information, Wasp creates a few entities behind the scenes. In this section, we will explain what entities are created and how they are connected.
### User Entity[](#user-entity "Direct link to User Entity")
When you want to add authentication to your app, you need to specify the user entity e.g. `User` in your Wasp file. This entity is a "business logic user" which represents a user of your app.
You can use this entity to store any information about the user that you want to store. For example, you might want to store the user's name or address. You can also use the user entity to define the relations between users and other entities in your app. For example, you might want to define a relation between a user and the tasks that they have created.
```
entity User {=psl id Int @id @default(autoincrement()) // Any other fields you want to store about the userpsl=}
```
You **own** the user entity and you can modify it as you wish. You can add new fields to it, remove fields from it, or change the type of the fields. You can also add new relations to it or remove existing relations from it.
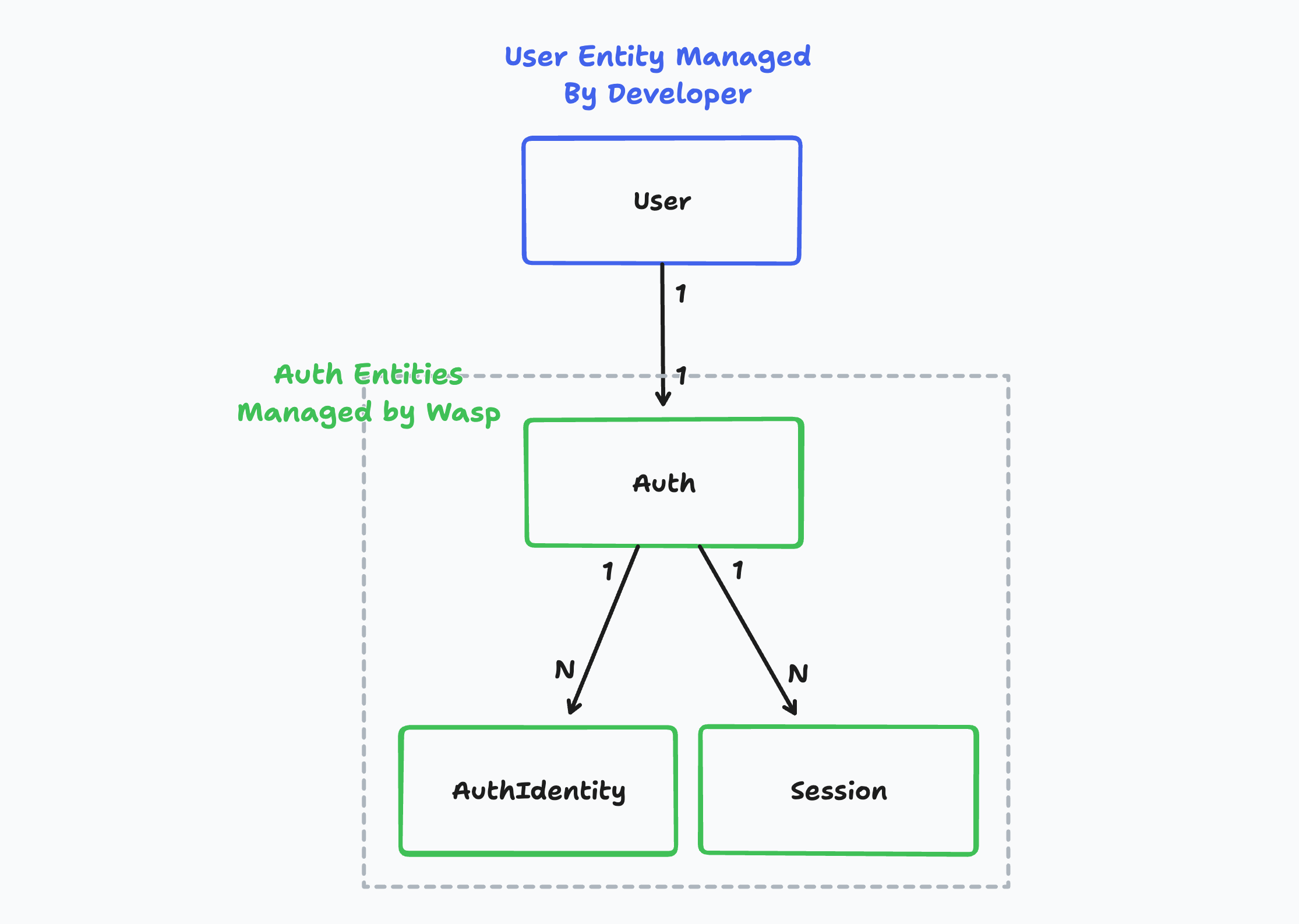
Auth Entities in a Wasp App
On the other hand, the `Auth`, `AuthIdentity` and `Session` entities are created behind the scenes and are used to store the user's login credentials. You as the developer don't need to care about this entity most of the time. Wasp **owns** these entities.
In the case you want to create a custom signup action, you will need to use the `Auth` and `AuthIdentity` entities directly.
### Example App Model[](#example-app-model "Direct link to Example App Model")
Let's imagine we created a simple tasks management app:
* The app has email and Google-based auth.
* Users can create tasks and see the tasks that they have created.
Let's look at how would that look in the database:
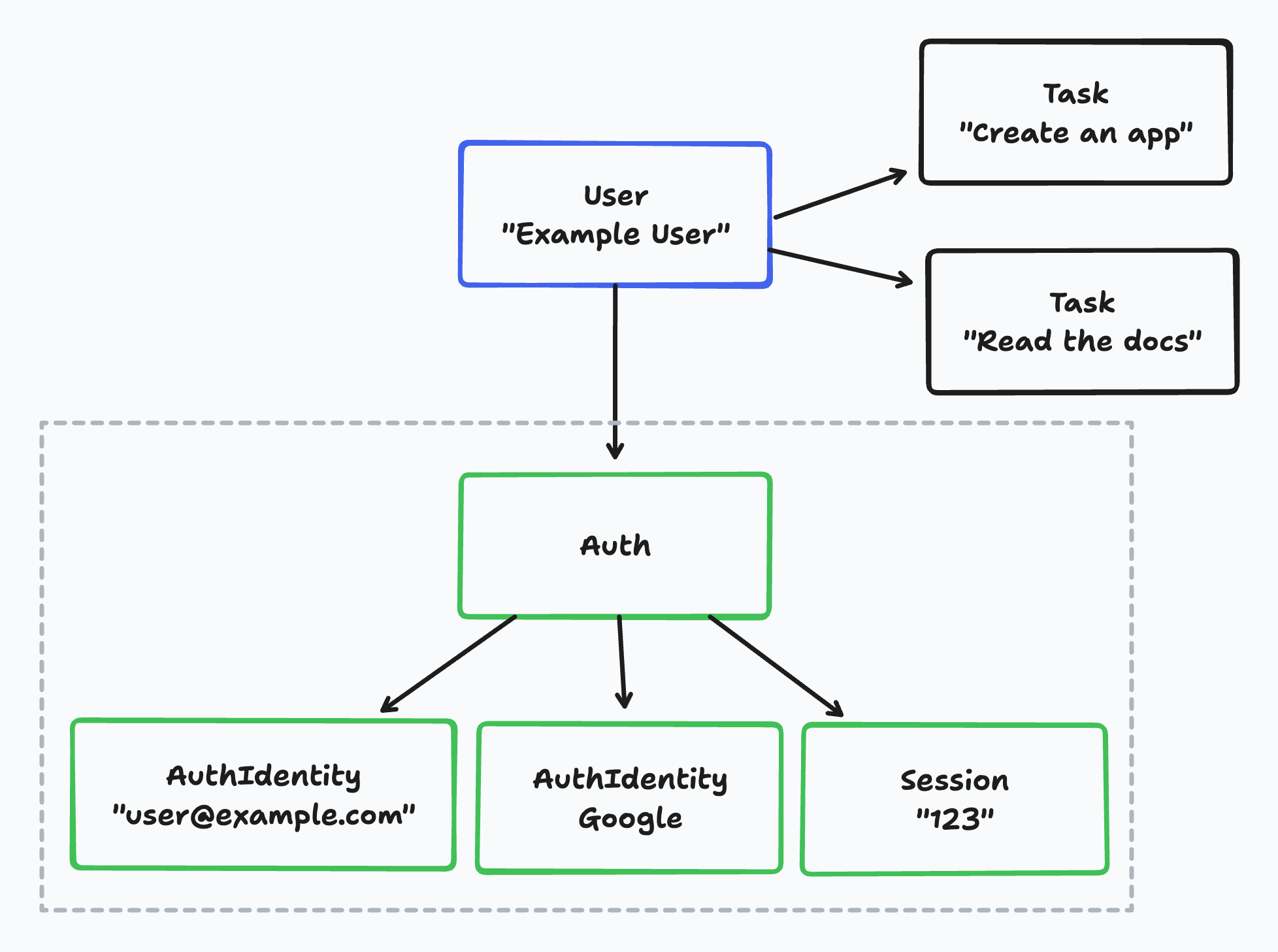
Example of Auth Entities
If we take a look at an example user in the database, we can see:
* The business logic user, `User` is connected to multiple `Task` entities.
* In this example, "Example User" has two tasks.
* The `User` is connected to exactly one `Auth` entity.
* Each `Auth` entity can have multiple `AuthIdentity` entities.
* In this example, the `Auth` entity has two `AuthIdentity` entities: one for the email-based auth and one for the Google-based auth.
* Each `Auth` entity can have multiple `Session` entities.
* In this example, the `Auth` entity has one `Session` entity.
Using multiple auth identities for a single user
Wasp currently doesn't support multiple auth identities for a single user. This means, for example, that a user can't have both an email-based auth identity and a Google-based auth identity. This is something we will add in the future with the introduction of the [account merging feature](https://github.com/wasp-lang/wasp/issues/954).
Account merging means that multiple auth identities can be merged into a single user account. For example, a user's email and Google identity can be merged into a single user account. Then the user can log in with either their email or Google account and they will be logged into the same account.
### `Auth` Entity internal[](#auth-entity- "Direct link to auth-entity-")
Wasp's internal `Auth` entity is used to connect the business logic user, `User` with the user's login credentials.
```
entity Auth {=psl id String @id @default(uuid()) userId Int? @unique // Wasp injects this relation on the User entity as well user User? @relation(fields: [userId], references: [id], onDelete: Cascade) identities AuthIdentity[] sessions Session[]psl=}
```
The `Auth` fields:
* `id` is a unique identifier of the `Auth` entity.
* `userId` is a foreign key to the `User` entity.
* It is used to connect the `Auth` entity with the business logic user.
* `user` is a relation to the `User` entity.
* This relation is injected on the `User` entity as well.
* `identities` is a relation to the `AuthIdentity` entity.
* `sessions` is a relation to the `Session` entity.
### `AuthIdentity` Entity internal[](#authidentity-entity- "Direct link to authidentity-entity-")
The `AuthIdentity` entity is used to store the user's login credentials for various authentication methods.
```
entity AuthIdentity {=psl providerName String providerUserId String providerData String @default("{}") authId String auth Auth @relation(fields: [authId], references: [id], onDelete: Cascade) @@id([providerName, providerUserId]) psl=}
```
The `AuthIdentity` fields:
* `providerName` is the name of the authentication provider.
* For example, `email` or `google`.
* `providerUserId` is the user's ID in the authentication provider.
* For example, the user's email or Google ID.
* `providerData` is a JSON string that contains additional data about the user from the authentication provider.
* For example, for password based auth, this field contains the user's hashed password.
* This field is a `String` and not a `Json` type because [Prisma doesn't support the `Json` type for SQLite](https://github.com/prisma/prisma/issues/3786).
* `authId` is a foreign key to the `Auth` entity.
* It is used to connect the `AuthIdentity` entity with the `Auth` entity.
* `auth` is a relation to the `Auth` entity.
### `Session` Entity internal[](#session-entity- "Direct link to session-entity-")
The `Session` entity is used to store the user's session information. It is used to keep the user logged in between page refreshes.
```
entity Session {=psl id String @id @unique expiresAt DateTime userId String auth Auth @relation(references: [id], fields: [userId], onDelete: Cascade) @@index([userId])psl=}
```
The `Session` fields:
* `id` is a unique identifier of the `Session` entity.
* `expiresAt` is the date when the session expires.
* `userId` is a foreign key to the `Auth` entity.
* It is used to connect the `Session` entity with the `Auth` entity.
* `auth` is a relation to the `Auth` entity.
## Accessing the Auth Fields[](#accessing-the-auth-fields "Direct link to Accessing the Auth Fields")
If you are looking to access the user's email or username in your code, you can do that by accessing the info about the user that is stored in the `AuthIdentity` entity.
Everywhere where Wasp gives you the `user` object, it also includes the `auth` relation with the `identities` relation. This means that you can access the auth identity info by using the `user.auth.identities` array.
To make things a bit easier for you, Wasp offers a few helper functions that you can use to access the auth identity info.
### `getEmail`[](#getemail "Direct link to getemail")
The `getEmail` helper returns the user's email or `null` if the user doesn't have an email auth identity.
* JavaScript
* TypeScript
src/MainPage.jsx
```
import { getEmail } from 'wasp/auth'const MainPage = ({ user }) => { const email = getEmail(user) // ...}
```
src/tasks.js
```
import { getEmail } from 'wasp/auth'export const createTask = async (args, context) => { const email = getEmail(context.user) // ...}
```
### `getUsername`[](#getusername "Direct link to getusername")
The `getUsername` helper returns the user's username or `null` if the user doesn't have a username auth identity.
* JavaScript
* TypeScript
src/MainPage.jsx
```
import { getUsername } from 'wasp/auth'const MainPage = ({ user }) => { const username = getUsername(user) // ...}
```
src/tasks.js
```
import { getUsername } from 'wasp/auth'export const createTask = async (args, context) => { const username = getUsername(context.user) // ...}
```
### `getFirstProviderUserId`[](#getfirstprovideruserid "Direct link to getfirstprovideruserid")
The `getFirstProviderUserId` helper returns the first user ID (e.g. `username` or `email`) that it finds for the user or `null` if it doesn't find any.
[As mentioned before](#authidentity-entity-), the `providerUserId` field is how providers identify our users. For example, the user's `username` in the case of the username auth or the user's `email` in the case of the email auth. This can be useful if you support multiple authentication methods and you need _any_ ID that identifies the user in your app.
* JavaScript
* TypeScript
src/MainPage.jsx
```
import { getFirstProviderUserId } from 'wasp/auth'const MainPage = ({ user }) => { const userId = getFirstProviderUserId(user) // ...}
```
src/tasks.js
```
import { getFirstProviderUserId } from 'wasp/auth'export const createTask = async (args, context) => { const userId = getFirstProviderUserId(context.user) // ...}
```
### `findUserIdentity`[](#finduseridentity "Direct link to finduseridentity")
You can find a specific auth identity by using the `findUserIdentity` helper function. This function takes a `user` and a `providerName` and returns the first `providerName` identity that it finds or `null` if it doesn't find any.
Possible provider names are:
* `email`
* `username`
* `google`
* `github`
This can be useful if you want to check if the user has a specific auth identity. For example, you might want to check if the user has an email auth identity or Google auth identity.
* JavaScript
* TypeScript
src/MainPage.jsx
```
import { findUserIdentity } from 'wasp/auth'const MainPage = ({ user }) => { const emailIdentity = findUserIdentity(user, 'email') const googleIdentity = findUserIdentity(user, 'google') if (emailIdentity) { // ... } else if (googleIdentity) { // ... } // ...}
```
src/tasks.js
```
import { findUserIdentity } from 'wasp/client/auth'export const createTask = async (args, context) => { const emailIdentity = findUserIdentity(context.user, 'email') const googleIdentity = findUserIdentity(context.user, 'google') if (emailIdentity) { // ... } else if (googleIdentity) { // ... } // ...}
```
## Custom Signup Action[](#custom-signup-action "Direct link to Custom Signup Action")
Let's take a look at how you can use the `Auth` and `AuthIdentity` entities to create custom login and signup actions. For example, you might want to create a custom signup action that creates a user in your app and also creates a user in a third-party service.
Custom Signup Examples
In the [Email](https://wasp-lang.dev/docs/auth/email#creating-a-custom-sign-up-action) section of the docs we give you an example for custom email signup and in the [Username & password](https://wasp-lang.dev/docs/auth/username-and-pass#2-creating-your-custom-sign-up-action) section of the docs we give you an example for custom username & password signup.
Below is a simplified version of a custom signup action which you probably wouldn't use in your app but it shows you how you can use the `Auth` and `AuthIdentity` entities to create a custom signup action.
* JavaScript
* TypeScript
main.wasp
```
// ...action customSignup { fn: import { signup } from "@src/auth/signup.js", entities: [User]}
```
src/auth/signup.js
```
import { createProviderId, sanitizeAndSerializeProviderData, createUser,} from 'wasp/server/auth'export const signup = async (args, { entities: { User } }) => { try { // Provider ID is a combination of the provider name and the provider user ID // And it is used to uniquely identify the user in your app const providerId = createProviderId('username', args.username) // sanitizeAndSerializeProviderData hashes the password and returns a JSON string const providerData = await sanitizeAndSerializeProviderData({ hashedPassword: args.password, }) await createUser( providerId, providerData, // Any additional data you want to store on the User entity {}, ) // This is equivalent to: // await User.create({ // data: { // auth: { // create: { // identities: { // create: { // providerName: 'username', // providerUserId: args.username // providerData, // }, // }, // } // }, // } // }) } catch (e) { return { success: false, message: e.message, } } // Your custom code after sign-up. // ... return { success: true, message: 'User created successfully', }}
```
You can use whichever method suits your needs better: either the `createUser` function or Prisma's `User.create` method. The `createUser` function is a bit more convenient to use because it hides some of the complexity. On the other hand, the `User.create` method gives you more control over the data that is stored in the `Auth` and `AuthIdentity` entities. | null | https://wasp-lang.dev/docs/auth/entities | Wasp supports multiple different authentication methods and for each method, we need to store different information about the user. For example, if you are using the Username & password authentication method, we need to store the user's username and password. On the other hand, if you are using the Email authentication method, you will need to store the user's email, password and for example, their email verification status. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecd7ddd762894-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:20 GMT | Tue, 23 Apr 2024 15:24:20 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=miT4RYmyTX1U1MquAQilbbLnwxwBFv0UoeckEGt21dqmIltV%2BfzKLUxxCuSTWNOeJqrbISc8DJkIXro95%2B3mjnRvOqm%2BlQfsmSTopy9CA4XJhWAFItUNr7DC0aFbRfWv"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 30af3cc56b3da83604a20bc733315ce645010807 | null | FBC6:53E77:3A82A4C:459F388:6627D04C | HIT | MISS | cache-iad-kiad7000074-IAD | S1713885260.481882,VS0,VE14 | null | null | en | og:image | https://wasp-lang.dev/docs/auth/entities | og:url | Auth Entities | Wasp | og:title | Wasp supports multiple different authentication methods and for each method, we need to store different information about the user. For example, if you are using the Username & password authentication method, we need to store the user's username and password. On the other hand, if you are using the Email authentication method, you will need to store the user's email, password and for example, their email verification status. | og:description | Auth Entities | Wasp | null | Auth Entities
Wasp supports multiple different authentication methods and for each method, we need to store different information about the user. For example, if you are using the Username & password authentication method, we need to store the user's username and password. On the other hand, if you are using the Email authentication method, you will need to store the user's email, password and for example, their email verification status.
Entities Explained
To store user information, Wasp creates a few entities behind the scenes. In this section, we will explain what entities are created and how they are connected.
User Entity
When you want to add authentication to your app, you need to specify the user entity e.g. User in your Wasp file. This entity is a "business logic user" which represents a user of your app.
You can use this entity to store any information about the user that you want to store. For example, you might want to store the user's name or address. You can also use the user entity to define the relations between users and other entities in your app. For example, you might want to define a relation between a user and the tasks that they have created.
entity User {=psl
id Int @id @default(autoincrement())
// Any other fields you want to store about the user
psl=}
You own the user entity and you can modify it as you wish. You can add new fields to it, remove fields from it, or change the type of the fields. You can also add new relations to it or remove existing relations from it.
Auth Entities in a Wasp App
On the other hand, the Auth, AuthIdentity and Session entities are created behind the scenes and are used to store the user's login credentials. You as the developer don't need to care about this entity most of the time. Wasp owns these entities.
In the case you want to create a custom signup action, you will need to use the Auth and AuthIdentity entities directly.
Example App Model
Let's imagine we created a simple tasks management app:
The app has email and Google-based auth.
Users can create tasks and see the tasks that they have created.
Let's look at how would that look in the database:
Example of Auth Entities
If we take a look at an example user in the database, we can see:
The business logic user, User is connected to multiple Task entities.
In this example, "Example User" has two tasks.
The User is connected to exactly one Auth entity.
Each Auth entity can have multiple AuthIdentity entities.
In this example, the Auth entity has two AuthIdentity entities: one for the email-based auth and one for the Google-based auth.
Each Auth entity can have multiple Session entities.
In this example, the Auth entity has one Session entity.
Using multiple auth identities for a single user
Wasp currently doesn't support multiple auth identities for a single user. This means, for example, that a user can't have both an email-based auth identity and a Google-based auth identity. This is something we will add in the future with the introduction of the account merging feature.
Account merging means that multiple auth identities can be merged into a single user account. For example, a user's email and Google identity can be merged into a single user account. Then the user can log in with either their email or Google account and they will be logged into the same account.
Auth Entity internal
Wasp's internal Auth entity is used to connect the business logic user, User with the user's login credentials.
entity Auth {=psl
id String @id @default(uuid())
userId Int? @unique
// Wasp injects this relation on the User entity as well
user User? @relation(fields: [userId], references: [id], onDelete: Cascade)
identities AuthIdentity[]
sessions Session[]
psl=}
The Auth fields:
id is a unique identifier of the Auth entity.
userId is a foreign key to the User entity.
It is used to connect the Auth entity with the business logic user.
user is a relation to the User entity.
This relation is injected on the User entity as well.
identities is a relation to the AuthIdentity entity.
sessions is a relation to the Session entity.
AuthIdentity Entity internal
The AuthIdentity entity is used to store the user's login credentials for various authentication methods.
entity AuthIdentity {=psl
providerName String
providerUserId String
providerData String @default("{}")
authId String
auth Auth @relation(fields: [authId], references: [id], onDelete: Cascade)
@@id([providerName, providerUserId])
psl=}
The AuthIdentity fields:
providerName is the name of the authentication provider.
For example, email or google.
providerUserId is the user's ID in the authentication provider.
For example, the user's email or Google ID.
providerData is a JSON string that contains additional data about the user from the authentication provider.
For example, for password based auth, this field contains the user's hashed password.
This field is a String and not a Json type because Prisma doesn't support the Json type for SQLite.
authId is a foreign key to the Auth entity.
It is used to connect the AuthIdentity entity with the Auth entity.
auth is a relation to the Auth entity.
Session Entity internal
The Session entity is used to store the user's session information. It is used to keep the user logged in between page refreshes.
entity Session {=psl
id String @id @unique
expiresAt DateTime
userId String
auth Auth @relation(references: [id], fields: [userId], onDelete: Cascade)
@@index([userId])
psl=}
The Session fields:
id is a unique identifier of the Session entity.
expiresAt is the date when the session expires.
userId is a foreign key to the Auth entity.
It is used to connect the Session entity with the Auth entity.
auth is a relation to the Auth entity.
Accessing the Auth Fields
If you are looking to access the user's email or username in your code, you can do that by accessing the info about the user that is stored in the AuthIdentity entity.
Everywhere where Wasp gives you the user object, it also includes the auth relation with the identities relation. This means that you can access the auth identity info by using the user.auth.identities array.
To make things a bit easier for you, Wasp offers a few helper functions that you can use to access the auth identity info.
getEmail
The getEmail helper returns the user's email or null if the user doesn't have an email auth identity.
JavaScript
TypeScript
src/MainPage.jsx
import { getEmail } from 'wasp/auth'
const MainPage = ({ user }) => {
const email = getEmail(user)
// ...
}
src/tasks.js
import { getEmail } from 'wasp/auth'
export const createTask = async (args, context) => {
const email = getEmail(context.user)
// ...
}
getUsername
The getUsername helper returns the user's username or null if the user doesn't have a username auth identity.
JavaScript
TypeScript
src/MainPage.jsx
import { getUsername } from 'wasp/auth'
const MainPage = ({ user }) => {
const username = getUsername(user)
// ...
}
src/tasks.js
import { getUsername } from 'wasp/auth'
export const createTask = async (args, context) => {
const username = getUsername(context.user)
// ...
}
getFirstProviderUserId
The getFirstProviderUserId helper returns the first user ID (e.g. username or email) that it finds for the user or null if it doesn't find any.
As mentioned before, the providerUserId field is how providers identify our users. For example, the user's username in the case of the username auth or the user's email in the case of the email auth. This can be useful if you support multiple authentication methods and you need any ID that identifies the user in your app.
JavaScript
TypeScript
src/MainPage.jsx
import { getFirstProviderUserId } from 'wasp/auth'
const MainPage = ({ user }) => {
const userId = getFirstProviderUserId(user)
// ...
}
src/tasks.js
import { getFirstProviderUserId } from 'wasp/auth'
export const createTask = async (args, context) => {
const userId = getFirstProviderUserId(context.user)
// ...
}
findUserIdentity
You can find a specific auth identity by using the findUserIdentity helper function. This function takes a user and a providerName and returns the first providerName identity that it finds or null if it doesn't find any.
Possible provider names are:
email
username
google
github
This can be useful if you want to check if the user has a specific auth identity. For example, you might want to check if the user has an email auth identity or Google auth identity.
JavaScript
TypeScript
src/MainPage.jsx
import { findUserIdentity } from 'wasp/auth'
const MainPage = ({ user }) => {
const emailIdentity = findUserIdentity(user, 'email')
const googleIdentity = findUserIdentity(user, 'google')
if (emailIdentity) {
// ...
} else if (googleIdentity) {
// ...
}
// ...
}
src/tasks.js
import { findUserIdentity } from 'wasp/client/auth'
export const createTask = async (args, context) => {
const emailIdentity = findUserIdentity(context.user, 'email')
const googleIdentity = findUserIdentity(context.user, 'google')
if (emailIdentity) {
// ...
} else if (googleIdentity) {
// ...
}
// ...
}
Custom Signup Action
Let's take a look at how you can use the Auth and AuthIdentity entities to create custom login and signup actions. For example, you might want to create a custom signup action that creates a user in your app and also creates a user in a third-party service.
Custom Signup Examples
In the Email section of the docs we give you an example for custom email signup and in the Username & password section of the docs we give you an example for custom username & password signup.
Below is a simplified version of a custom signup action which you probably wouldn't use in your app but it shows you how you can use the Auth and AuthIdentity entities to create a custom signup action.
JavaScript
TypeScript
main.wasp
// ...
action customSignup {
fn: import { signup } from "@src/auth/signup.js",
entities: [User]
}
src/auth/signup.js
import {
createProviderId,
sanitizeAndSerializeProviderData,
createUser,
} from 'wasp/server/auth'
export const signup = async (args, { entities: { User } }) => {
try {
// Provider ID is a combination of the provider name and the provider user ID
// And it is used to uniquely identify the user in your app
const providerId = createProviderId('username', args.username)
// sanitizeAndSerializeProviderData hashes the password and returns a JSON string
const providerData = await sanitizeAndSerializeProviderData({
hashedPassword: args.password,
})
await createUser(
providerId,
providerData,
// Any additional data you want to store on the User entity
{},
)
// This is equivalent to:
// await User.create({
// data: {
// auth: {
// create: {
// identities: {
// create: {
// providerName: 'username',
// providerUserId: args.username
// providerData,
// },
// },
// }
// },
// }
// })
} catch (e) {
return {
success: false,
message: e.message,
}
}
// Your custom code after sign-up.
// ...
return {
success: true,
message: 'User created successfully',
}
}
You can use whichever method suits your needs better: either the createUser function or Prisma's User.create method. The createUser function is a bit more convenient to use because it hides some of the complexity. On the other hand, the User.create method gives you more control over the data that is stored in the Auth and AuthIdentity entities. | https://wasp-lang.dev/docs/auth/entities |
|
1 | null | 2024-04-23T15:14:23.059Z | https://wasp-lang.dev/docs/project/starter-templates | https://wasp-lang.dev/docs | http | ## Starter Templates
We created a few starter templates to help you get started with Wasp. Check out the list [below](#available-templates).
## Using a Template[](#using-a-template "Direct link to Using a Template")
Run `wasp new` to run the interactive mode for creating a new Wasp project.
It will ask you for the project name, and then for the template to use:
```
$ wasp newEnter the project name (e.g. my-project) ▸ MyFirstProjectChoose a starter template[1] basic (default) Simple starter template with a single page.[2] todo-ts Simple but well-rounded Wasp app implemented with Typescript & full-stack type safety.[3] saas Everything a SaaS needs! Comes with Auth, ChatGPT API, Tailwind, Stripe payments and more. Check out https://opensaas.sh/ for more details.[4] embeddings Comes with code for generating vector embeddings and performing vector similarity search.[5] ai-generated 🤖 Describe an app in a couple of sentences and have Wasp AI generate initial code for you. (experimental) ▸ 1🐝 --- Creating your project from the "basic" template... -------------------------Created new Wasp app in ./MyFirstProject directory!To run your new app, do: cd MyFirstProject wasp db start
```
## Available Templates[](#available-templates "Direct link to Available Templates")
When you have a good idea for a new product, you don't want to waste your time on setting up common things like authentication, database, etc. That's why we created a few starter templates to help you get started with Wasp.
### OpenSaaS.sh template[](#opensaassh-template "Direct link to OpenSaaS.sh template")
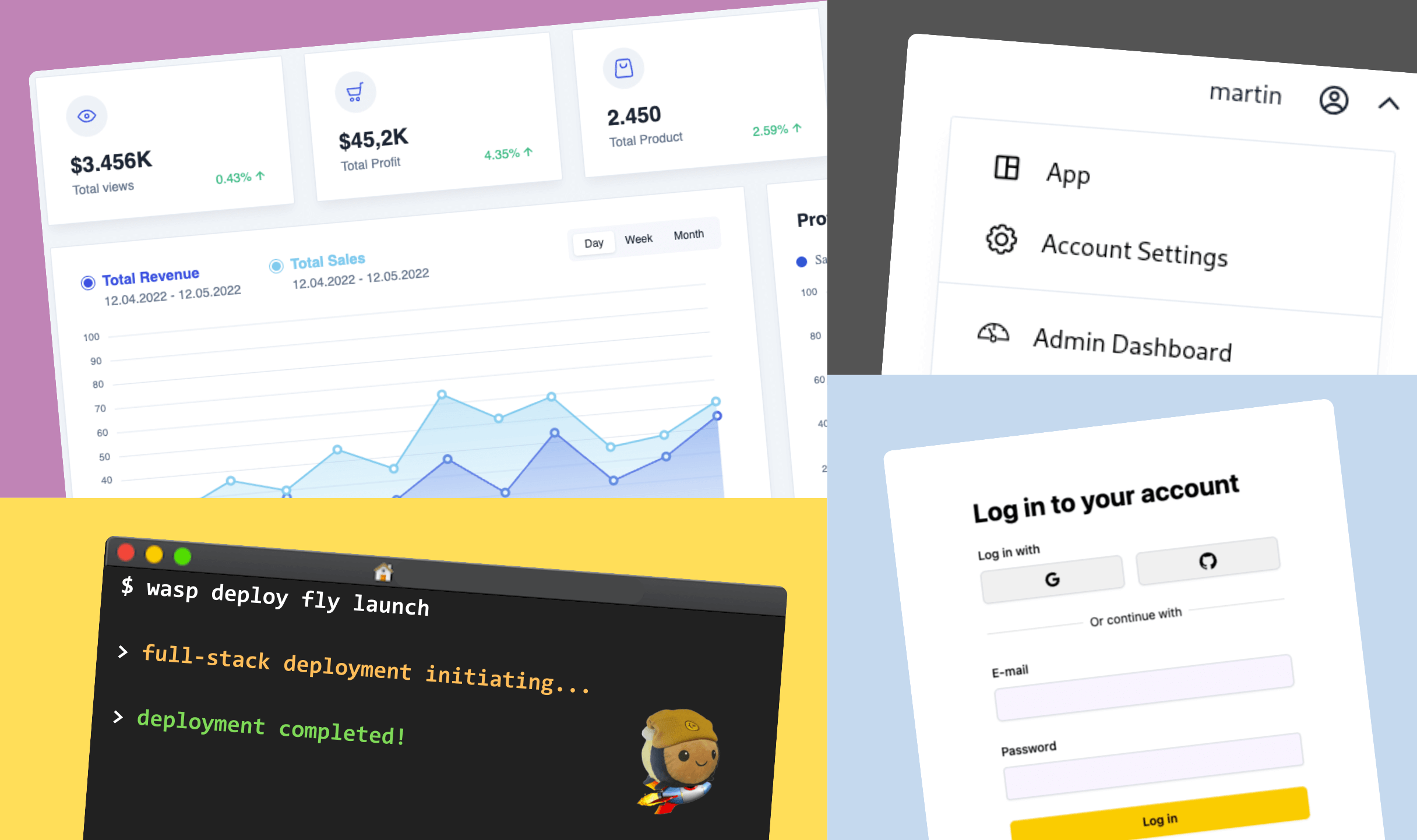
Everything a SaaS needs! Comes with Auth, ChatGPT API, Tailwind, Stripe payments and more. Check out [https://opensaas.sh/](https://opensaas.sh/) for more details.
**Features:** Stripe Payments, OpenAI GPT API, Google Auth, SendGrid, Tailwind, & Cron Jobs
Use this template:
```
wasp new <project-name> -t saas
```
### Vector Similarity Search Template[](#vector-similarity-search-template "Direct link to Vector Similarity Search Template")
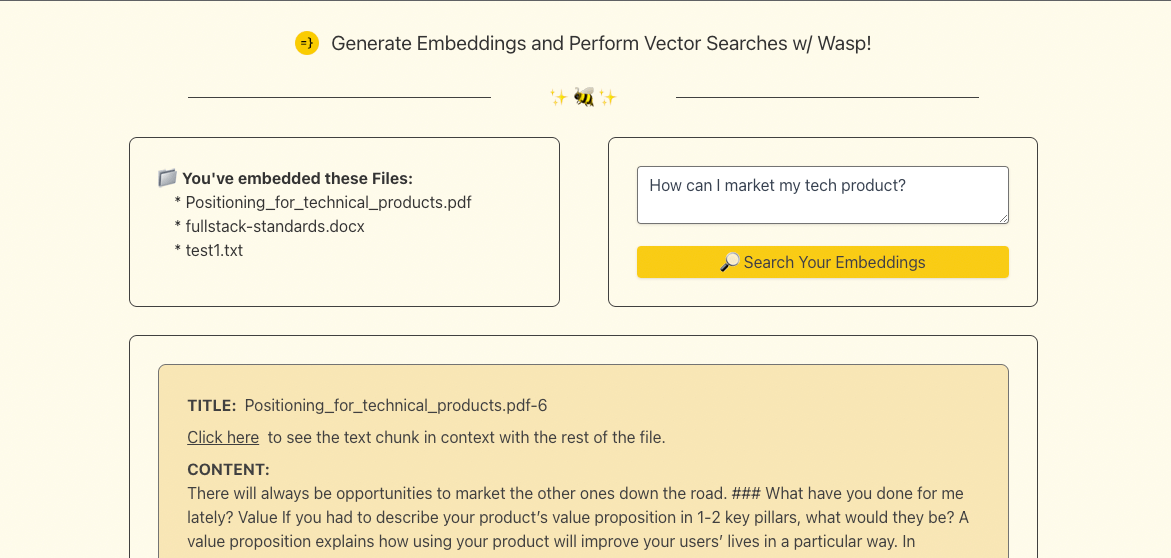
A template for generating embeddings and performing vector similarity search on your text data!
**Features:** Embeddings & vector similarity search, OpenAI Embeddings API, Vector DB (Pinecone), Tailwind, Full-stack Type Safety
Use this template:
```
wasp new <project-name> -t embeddings
```
### Todo App w/ Typescript[](#todo-app-w-typescript "Direct link to Todo App w/ Typescript")
A simple Todo App with Typescript and Full-stack Type Safety.
**Features:** Auth (username/password), Full-stack Type Safety
Use this template:
```
wasp new <project-name> -t todo-ts
```
### AI Generated Starter 🤖[](#ai-generated-starter- "Direct link to AI Generated Starter 🤖")
Using the same tech as used on [https://usemage.ai/](https://usemage.ai/), Wasp generates your custom starter template based on your project description. It will automatically generate your data model, auth, queries, actions and React pages.
_You will need to provide your own OpenAI API key to be able to use this template._
**Features:** Generated using OpenAI's GPT models, Auth (username/password), Queries, Actions, Pages, Full-stack Type Safety | null | https://wasp-lang.dev/docs/project/starter-templates | We created a few starter templates to help you get started with Wasp. Check out the list below. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecd8d99ba5ae6-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:23 GMT | Tue, 23 Apr 2024 15:24:23 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=g4gebaou890RFqqo%2BZ%2BYOeRcbVnG2CC5GFZVmqCqGV28VF5WhGgdMeQvM7jc4ZrqYCcsResok3PAKmRN8NEPPR51rvkbmbblMKo%2BKmwLjSzDfCC53%2BmeAk36RDu1IAyr"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 5d03c0305ace367a735ddb1434270307d89185e4 | null | 96DE:35D1BB:3B37AB0:4654856:6627D04E | HIT | MISS | cache-iad-kiad7000061-IAD | S1713885263.023469,VS0,VE20 | null | null | en | og:image | https://wasp-lang.dev/docs/project/starter-templates | og:url | Starter Templates | Wasp | og:title | We created a few starter templates to help you get started with Wasp. Check out the list below. | og:description | Starter Templates | Wasp | null | Starter Templates
We created a few starter templates to help you get started with Wasp. Check out the list below.
Using a Template
Run wasp new to run the interactive mode for creating a new Wasp project.
It will ask you for the project name, and then for the template to use:
$ wasp new
Enter the project name (e.g. my-project) ▸ MyFirstProject
Choose a starter template
[1] basic (default)
Simple starter template with a single page.
[2] todo-ts
Simple but well-rounded Wasp app implemented with Typescript & full-stack type safety.
[3] saas
Everything a SaaS needs! Comes with Auth, ChatGPT API, Tailwind, Stripe payments and more. Check out https://opensaas.sh/ for more details.
[4] embeddings
Comes with code for generating vector embeddings and performing vector similarity search.
[5] ai-generated
🤖 Describe an app in a couple of sentences and have Wasp AI generate initial code for you. (experimental)
▸ 1
🐝 --- Creating your project from the "basic" template... -------------------------
Created new Wasp app in ./MyFirstProject directory!
To run your new app, do:
cd MyFirstProject
wasp db start
Available Templates
When you have a good idea for a new product, you don't want to waste your time on setting up common things like authentication, database, etc. That's why we created a few starter templates to help you get started with Wasp.
OpenSaaS.sh template
Everything a SaaS needs! Comes with Auth, ChatGPT API, Tailwind, Stripe payments and more. Check out https://opensaas.sh/ for more details.
Features: Stripe Payments, OpenAI GPT API, Google Auth, SendGrid, Tailwind, & Cron Jobs
Use this template:
wasp new <project-name> -t saas
Vector Similarity Search Template
A template for generating embeddings and performing vector similarity search on your text data!
Features: Embeddings & vector similarity search, OpenAI Embeddings API, Vector DB (Pinecone), Tailwind, Full-stack Type Safety
Use this template:
wasp new <project-name> -t embeddings
Todo App w/ Typescript
A simple Todo App with Typescript and Full-stack Type Safety.
Features: Auth (username/password), Full-stack Type Safety
Use this template:
wasp new <project-name> -t todo-ts
AI Generated Starter 🤖
Using the same tech as used on https://usemage.ai/, Wasp generates your custom starter template based on your project description. It will automatically generate your data model, auth, queries, actions and React pages.
You will need to provide your own OpenAI API key to be able to use this template.
Features: Generated using OpenAI's GPT models, Auth (username/password), Queries, Actions, Pages, Full-stack Type Safety | https://wasp-lang.dev/docs/project/starter-templates |
|
1 | null | 2024-04-23T15:14:24.799Z | https://wasp-lang.dev/docs/project/customizing-app | https://wasp-lang.dev/docs | http | Version: 0.13.0
## Customizing the App
Each Wasp project can have only one `app` type declaration. It is used to configure your app and its components.
```
app todoApp { wasp: { version: "^0.13.0" }, title: "ToDo App", head: [ "<link rel=\"stylesheet\" href=\"https://fonts.googleapis.com/css?family=Roboto:300,400,500&display=swap\" />" ]}
```
We'll go through some common customizations you might want to do to your app. For more details on each of the fields, check out the [API Reference](#api-reference).
### Changing the App Title[](#changing-the-app-title "Direct link to Changing the App Title")
You may want to change the title of your app, which appears in the browser tab, next to the favicon. You can change it by changing the `title` field of your `app` declaration:
```
app myApp { wasp: { version: "^0.13.0" }, title: "BookFace"}
```
### Adding Additional Lines to the Head[](#adding-additional-lines-to-the-head "Direct link to Adding Additional Lines to the Head")
If you are looking to add additional style sheets or scripts to your app, you can do so by adding them to the `head` field of your `app` declaration.
An example of adding extra style sheets and scripts:
```
app myApp { wasp: { version: "^0.13.0" }, title: "My App", head: [ // optional "<link rel=\"stylesheet\" href=\"https://fonts.googleapis.com/css?family=Roboto:300,400,500&display=swap\" />", "<script src=\"https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.9.3/Chart.min.js\"></script>", "<meta name=\"viewport\" content=\"minimum-scale=1, initial-scale=1, width=device-width\" />" ]}
```
## API Reference[](#api-reference "Direct link to API Reference")
```
app todoApp { wasp: { version: "^0.13.0" }, title: "ToDo App", head: [ "<link rel=\"stylesheet\" href=\"https://fonts.googleapis.com/css?family=Roboto:300,400,500&display=swap\" />" ], auth: { // ... }, client: { // ... }, server: { // ... }, db: { // ... }, emailSender: { // ... }, webSocket: { // ... }}
```
The `app` declaration has the following fields:
* `wasp: dict` required Wasp compiler configuration. It is a dictionary with a single field:
* `version: string` required
The version specifies which versions of Wasp are compatible with the app. It should contain a valid [SemVer range](https://github.com/npm/node-semver#ranges)
info
For now, the version field only supports caret ranges (i.e., `^x.y.z`). Support for the full specification will come in a future version of Wasp
* `title: string` required
Title of your app. It will appear in the browser tab, next to the favicon.
* `head: [string]`
List of additional lines (e.g. `<link>` or `<script>` tags) to be included in the `<head>` of your HTML document.
The rest of the fields are covered in dedicated sections of the docs:
* `auth: dict`
Authentication configuration. Read more in the [authentication section](https://wasp-lang.dev/docs/auth/overview) of the docs.
* `client: dict`
Configuration for the client side of your app. Read more in the [client configuration section](https://wasp-lang.dev/docs/project/client-config) of the docs.
* `server: dict`
Configuration for the server side of your app. Read more in the [server configuration section](https://wasp-lang.dev/docs/project/server-config) of the docs.
* `db: dict`
Database configuration. Read more in the [database configuration section](https://wasp-lang.dev/docs/data-model/backends) of the docs.
* `emailSender: dict`
Email sender configuration. Read more in the [email sending section](https://wasp-lang.dev/docs/advanced/email) of the docs.
* `webSocket: dict`
WebSocket configuration. Read more in the [WebSocket section](https://wasp-lang.dev/docs/advanced/web-sockets) of the docs. | null | https://wasp-lang.dev/docs/project/customizing-app | Each Wasp project can have only one app type declaration. It is used to configure your app and its components. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecd989f332021-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:24 GMT | Tue, 23 Apr 2024 15:24:24 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=vwakNL%2FSbpKjFvysFooNdmzjsnA%2F%2FF%2F6A5U614jlGa2miz2zGVL7SAsr%2FiTXEDoIhREoJMEPxdAYBz0OpA4yOfeMHvilpxqx8oV6op8%2BZCasUybcwSMolaVFLQhhyOTJ"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | beb85d14aa1a0a07eb3a124f6f390c7db0f33a07 | null | 6538:2134BE:3C513E3:476BED2:6627D050 | HIT | MISS | cache-iad-kiad7000042-IAD | S1713885265.761534,VS0,VE22 | null | null | en | og:image | https://wasp-lang.dev/docs/project/customizing-app | og:url | Customizing the App | Wasp | og:title | Each Wasp project can have only one app type declaration. It is used to configure your app and its components. | og:description | Customizing the App | Wasp | null | Version: 0.13.0
Customizing the App
Each Wasp project can have only one app type declaration. It is used to configure your app and its components.
app todoApp {
wasp: {
version: "^0.13.0"
},
title: "ToDo App",
head: [
"<link rel=\"stylesheet\" href=\"https://fonts.googleapis.com/css?family=Roboto:300,400,500&display=swap\" />"
]
}
We'll go through some common customizations you might want to do to your app. For more details on each of the fields, check out the API Reference.
Changing the App Title
You may want to change the title of your app, which appears in the browser tab, next to the favicon. You can change it by changing the title field of your app declaration:
app myApp {
wasp: {
version: "^0.13.0"
},
title: "BookFace"
}
Adding Additional Lines to the Head
If you are looking to add additional style sheets or scripts to your app, you can do so by adding them to the head field of your app declaration.
An example of adding extra style sheets and scripts:
app myApp {
wasp: {
version: "^0.13.0"
},
title: "My App",
head: [ // optional
"<link rel=\"stylesheet\" href=\"https://fonts.googleapis.com/css?family=Roboto:300,400,500&display=swap\" />",
"<script src=\"https://cdnjs.cloudflare.com/ajax/libs/Chart.js/2.9.3/Chart.min.js\"></script>",
"<meta name=\"viewport\" content=\"minimum-scale=1, initial-scale=1, width=device-width\" />"
]
}
API Reference
app todoApp {
wasp: {
version: "^0.13.0"
},
title: "ToDo App",
head: [
"<link rel=\"stylesheet\" href=\"https://fonts.googleapis.com/css?family=Roboto:300,400,500&display=swap\" />"
],
auth: {
// ...
},
client: {
// ...
},
server: {
// ...
},
db: {
// ...
},
emailSender: {
// ...
},
webSocket: {
// ...
}
}
The app declaration has the following fields:
wasp: dict required Wasp compiler configuration. It is a dictionary with a single field:
version: string required
The version specifies which versions of Wasp are compatible with the app. It should contain a valid SemVer range
info
For now, the version field only supports caret ranges (i.e., ^x.y.z). Support for the full specification will come in a future version of Wasp
title: string required
Title of your app. It will appear in the browser tab, next to the favicon.
head: [string]
List of additional lines (e.g. <link> or <script> tags) to be included in the <head> of your HTML document.
The rest of the fields are covered in dedicated sections of the docs:
auth: dict
Authentication configuration. Read more in the authentication section of the docs.
client: dict
Configuration for the client side of your app. Read more in the client configuration section of the docs.
server: dict
Configuration for the server side of your app. Read more in the server configuration section of the docs.
db: dict
Database configuration. Read more in the database configuration section of the docs.
emailSender: dict
Email sender configuration. Read more in the email sending section of the docs.
webSocket: dict
WebSocket configuration. Read more in the WebSocket section of the docs. | https://wasp-lang.dev/docs/project/customizing-app |
|
1 | null | 2024-04-23T15:14:31.939Z | https://wasp-lang.dev/docs/project/server-config | https://wasp-lang.dev/docs | http | ## Server Config
You can configure the behavior of the server via the `server` field of `app` declaration:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { title: "My app", // ... server: { setupFn: import { mySetupFunction } from "@src/myServerSetupCode.js", middlewareConfigFn: import { myMiddlewareConfigFn } from "@src/myServerSetupCode.js" }}
```
## Setup Function[](#setup-function "Direct link to Setup Function")
### Adding a Custom Route[](#adding-a-custom-route "Direct link to Adding a Custom Route")
As an example, adding a custom route would look something like:
* JavaScript
* TypeScript
src/myServerSetupCode.ts
```
export const mySetupFunction = async ({ app }) => { addCustomRoute(app)}function addCustomRoute(app) { app.get('/customRoute', (_req, res) => { res.send('I am a custom route') })}
```
### Storing Some Values for Later Use[](#storing-some-values-for-later-use "Direct link to Storing Some Values for Later Use")
In case you want to store some values for later use, or to be accessed by the [Operations](https://wasp-lang.dev/docs/data-model/operations/overview) you do that in the `setupFn` function.
Dummy example of such function and its usage:
* JavaScript
* TypeScript
src/myServerSetupCode.js
```
let someResource = undefinedexport const mySetupFunction = async () => { // Let's pretend functions setUpSomeResource and startSomeCronJob // are implemented below or imported from another file. someResource = await setUpSomeResource() startSomeCronJob()}export const getSomeResource = () => someResource
```
src/queries.js
```
import { getSomeResource } from './myServerSetupCode.js'...export const someQuery = async (args, context) => { const someResource = getSomeResource() return queryDataFromSomeResource(args, someResource)}
```
note
The recommended way is to put the variable in the same module where you defined the setup function and then expose additional functions for reading those values, which you can then import directly from Operations and use.
This effectively turns your module into a singleton whose construction is performed on server start.
Read more about [server setup function](#setupfn-extimport) below.
## Middleware Config Function[](#middleware-config-function "Direct link to Middleware Config Function")
You can configure the global middleware via the `middlewareConfigFn`. This will modify the middleware stack for all operations and APIs.
Read more about [middleware config function](#middlewareconfigfn-extimport) below.
## API Reference[](#api-reference "Direct link to API Reference")
* JavaScript
* TypeScript
main.wasp
```
app MyApp { title: "My app", // ... server: { setupFn: import { mySetupFunction } from "@src/myServerSetupCode.js", middlewareConfigFn: import { myMiddlewareConfigFn } from "@src/myServerSetupCode.js" }}
```
`app.server` is a dictionary with the following fields:
* #### `setupFn: ExtImport`[](#setupfn-extimport "Direct link to setupfn-extimport")
`setupFn` declares a function that will be executed on server start. This function is expected to be async and will be awaited before the server starts accepting any requests.
It allows you to do any custom setup, e.g. setting up additional database/websockets or starting cron/scheduled jobs.
The `setupFn` function receives the `express.Application` and the `http.Server` instances as part of its context. They can be useful for setting up any custom server logic.
* JavaScript
* TypeScript
src/myServerSetupCode.js
```
export const mySetupFunction = async () => { await setUpSomeResource()}
```
* #### `middlewareConfigFn: ExtImport`[](#middlewareconfigfn-extimport "Direct link to middlewareconfigfn-extimport")
The import statement to an Express middleware config function. This is a global modification affecting all operations and APIs. See more in the [configuring middleware section](https://wasp-lang.dev/docs/advanced/middleware-config#1-customize-global-middleware). | null | https://wasp-lang.dev/docs/project/server-config | You can configure the behavior of the server via the server field of app declaration: | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecdc54c182414-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:31 GMT | Tue, 23 Apr 2024 15:24:31 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=5fOkykkONLCPrrZu0HE1W8b%2FVugAKoFr4TVzEKEoGT6OyV621PH%2BuRo6T1MKOqiAoZT8y5tAn5FW6Wn2U8Le%2BlqCBQ9%2FtGG9tiy3Yt7yd5t8WxhXHdKh1jByUq8G2k1z"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 6c5208a5611b0f920715847d975fa68024afca88 | null | B9F8:2134BE:3C519AC:476C56D:6627D057 | HIT | MISS | cache-iad-kiad7000042-IAD | S1713885272.901220,VS0,VE12 | null | null | en | og:image | https://wasp-lang.dev/docs/project/server-config | og:url | Server Config | Wasp | og:title | You can configure the behavior of the server via the server field of app declaration: | og:description | Server Config | Wasp | null | Server Config
You can configure the behavior of the server via the server field of app declaration:
JavaScript
TypeScript
main.wasp
app MyApp {
title: "My app",
// ...
server: {
setupFn: import { mySetupFunction } from "@src/myServerSetupCode.js",
middlewareConfigFn: import { myMiddlewareConfigFn } from "@src/myServerSetupCode.js"
}
}
Setup Function
Adding a Custom Route
As an example, adding a custom route would look something like:
JavaScript
TypeScript
src/myServerSetupCode.ts
export const mySetupFunction = async ({ app }) => {
addCustomRoute(app)
}
function addCustomRoute(app) {
app.get('/customRoute', (_req, res) => {
res.send('I am a custom route')
})
}
Storing Some Values for Later Use
In case you want to store some values for later use, or to be accessed by the Operations you do that in the setupFn function.
Dummy example of such function and its usage:
JavaScript
TypeScript
src/myServerSetupCode.js
let someResource = undefined
export const mySetupFunction = async () => {
// Let's pretend functions setUpSomeResource and startSomeCronJob
// are implemented below or imported from another file.
someResource = await setUpSomeResource()
startSomeCronJob()
}
export const getSomeResource = () => someResource
src/queries.js
import { getSomeResource } from './myServerSetupCode.js'
...
export const someQuery = async (args, context) => {
const someResource = getSomeResource()
return queryDataFromSomeResource(args, someResource)
}
note
The recommended way is to put the variable in the same module where you defined the setup function and then expose additional functions for reading those values, which you can then import directly from Operations and use.
This effectively turns your module into a singleton whose construction is performed on server start.
Read more about server setup function below.
Middleware Config Function
You can configure the global middleware via the middlewareConfigFn. This will modify the middleware stack for all operations and APIs.
Read more about middleware config function below.
API Reference
JavaScript
TypeScript
main.wasp
app MyApp {
title: "My app",
// ...
server: {
setupFn: import { mySetupFunction } from "@src/myServerSetupCode.js",
middlewareConfigFn: import { myMiddlewareConfigFn } from "@src/myServerSetupCode.js"
}
}
app.server is a dictionary with the following fields:
setupFn: ExtImport
setupFn declares a function that will be executed on server start. This function is expected to be async and will be awaited before the server starts accepting any requests.
It allows you to do any custom setup, e.g. setting up additional database/websockets or starting cron/scheduled jobs.
The setupFn function receives the express.Application and the http.Server instances as part of its context. They can be useful for setting up any custom server logic.
JavaScript
TypeScript
src/myServerSetupCode.js
export const mySetupFunction = async () => {
await setUpSomeResource()
}
middlewareConfigFn: ExtImport
The import statement to an Express middleware config function. This is a global modification affecting all operations and APIs. See more in the configuring middleware section. | https://wasp-lang.dev/docs/project/server-config |
|
1 | 200 | 2024-04-23T15:14:29.818Z | https://wasp-lang.dev/docs/project/client-config | https://wasp-lang.dev/docs | browser | ## Client Config
You can configure the client using the `client` field inside the `app` declaration:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { title: "My app", // ... client: { rootComponent: import Root from "@src/Root.jsx", setupFn: import mySetupFunction from "@src/myClientSetupCode.js" }}
```
## Root Component[](#root-component "Direct link to Root Component")
Wasp gives you the option to define a "wrapper" component for your React app.
It can be used for a variety of purposes, but the most common ones are:
* Defining a common layout for your application.
* Setting up various providers that your application needs.
### Defining a Common Layout[](#defining-a-common-layout "Direct link to Defining a Common Layout")
Let's define a common layout for your application:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { title: "My app", // ... client: { rootComponent: import Root from "@src/Root.jsx", }}
```
src/Root.jsx
```
export default function Root({ children }) { return ( <div> <header> <h1>My App</h1> </header> {children} <footer> <p>My App footer</p> </footer> </div> )}
```
### Setting up a Provider[](#setting-up-a-provider "Direct link to Setting up a Provider")
This is how to set up various providers that your application needs:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { title: "My app", // ... client: { rootComponent: import Root from "@src/Root.jsx", }}
```
src/Root.jsx
```
import store from './store'import { Provider } from 'react-redux'export default function Root({ children }) { return <Provider store={store}>{children}</Provider>}
```
As long as you render the children, you can do whatever you want in your root component.
Read more about the root component in the [API Reference](#rootcomponent-extimport).
## Setup Function[](#setup-function "Direct link to Setup Function")
`setupFn` declares a function that Wasp executes on the client before everything else.
### Running Some Code[](#running-some-code "Direct link to Running Some Code")
We can run any code we want in the setup function.
For example, here's a setup function that logs a message every hour:
* JavaScript
* TypeScript
src/myClientSetupCode.js
```
export default async function mySetupFunction() { let count = 1 setInterval( () => console.log(`You have been online for ${count++} hours.`), 1000 * 60 * 60 )}
```
### Overriding Default Behaviour for Queries[](#overriding-default-behaviour-for-queries "Direct link to Overriding Default Behaviour for Queries")
info
You can change the options for a **single** Query using the `options` object, as described [here](https://wasp-lang.dev/docs/data-model/operations/queries#the-usequery-hook-1).
Wasp's `useQuery` hook uses `react-query`'s `useQuery` hook under the hood. Since `react-query` comes configured with aggressive but sane default options, you most likely won't have to change those defaults for all Queries.
If you do need to change the global defaults, you can do so inside the client setup function.
Wasp exposes a `configureQueryClient` hook that lets you configure _react-query_'s `QueryClient` object:
* JavaScript
* TypeScript
src/myClientSetupCode.js
```
import { configureQueryClient } from 'wasp/client/operations'export default async function mySetupFunction() { // ... some setup configureQueryClient({ defaultOptions: { queries: { staleTime: Infinity, }, }, }) // ... some more setup}
```
Make sure to pass in an object expected by the `QueryClient`'s constructor, as explained in [react-query's docs](https://tanstack.com/query/v4/docs/react/reference/QueryClient).
Read more about the setup function in the [API Reference](#setupfn-extimport).
## Base Directory[](#base-directory "Direct link to Base Directory")
If you need to serve the client from a subdirectory, you can use the `baseDir` option:
main.wasp
```
app MyApp { title: "My app", // ... client: { baseDir: "/my-app", }}
```
This means that if you serve your app from `https://example.com/my-app`, the router will work correctly, and all the assets will be served from `https://example.com/my-app`.
Setting the correct env variable
If you set the `baseDir` option, make sure that the `WASP_WEB_CLIENT_URL` env variable also includes that base directory.
For example, if you are serving your app from `https://example.com/my-app`, the `WASP_WEB_CLIENT_URL` should be also set to `https://example.com/my-app`, and not just `https://example.com`.
## API Reference[](#api-reference "Direct link to API Reference")
* JavaScript
* TypeScript
main.wasp
```
app MyApp { title: "My app", // ... client: { rootComponent: import Root from "@src/Root.jsx", setupFn: import mySetupFunction from "@src/myClientSetupCode.js" }}
```
Client has the following options:
* #### `rootComponent: ExtImport`[](#rootcomponent-extimport "Direct link to rootcomponent-extimport")
`rootComponent` defines the root component of your client application. It is expected to be a React component, and Wasp will use it to wrap your entire app. It must render its children, which are the actual pages of your application.
Here's an example of a root component that both sets up a provider and renders a custom layout:
* JavaScript
* TypeScript
src/Root.jsx
```
import store from './store'import { Provider } from 'react-redux'export default function Root({ children }) { return ( <Provider store={store}> <Layout>{children}</Layout> </Provider> )}function Layout({ children }) { return ( <div> <header> <h1>My App</h1> </header> {children} <footer> <p>My App footer</p> </footer> </div> )}
```
* #### `setupFn: ExtImport`[](#setupfn-extimport "Direct link to setupfn-extimport")
You can use this function to perform any custom setup (e.g., setting up client-side periodic jobs).
* JavaScript
* TypeScript
src/myClientSetupCode.js
```
export default async function mySetupFunction() { // Run some code}
```
* #### `baseDir: String`[](#basedir-string "Direct link to basedir-string")
If you need to serve the client from a subdirectory, you can use the `baseDir` option.
If you set `baseDir` to `/my-app` for example, that will make Wasp set the `basename` prop of the `Router` to `/my-app`. It will also set the `base` option of the Vite config to `/my-app`.
This means that if you serve your app from `https://example.com/my-app`, the router will work correctly, and all the assets will be served from `https://example.com/my-app`.
Setting the correct env variable
If you set the `baseDir` option, make sure that the `WASP_WEB_CLIENT_URL` env variable also includes that base directory.
For example, if you are serving your app from `https://example.com/my-app`, the `WASP_WEB_CLIENT_URL` should be also set to `https://example.com/my-app`, and not just `https://example.com`. | null | https://wasp-lang.dev/docs/project/client-config | You can configure the client using the client field inside the app declaration: | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecda93cae4bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:27 GMT | Tue, 23 Apr 2024 15:24:27 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=3Xo22nxa5vNNzA9hQK4ZEjXX6Hr%2BoaM6S48%2FlCY9%2BLrDDK6oPMuFqLr%2BHvGt965QIK%2BLOVLRbRNfdxhZC%2FoFo7XtQCDgT6Vrhsw6omgG7ST%2F1Kav60yrFv42GtKNFDm%2B"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | cccb6963dd45af61bfc9193de16f7979b6658cf4 | h2 | CC0E:16D7:483B02:554D13:6627D053 | HIT | MISS | cache-nyc-kteb1890087-NYC | S1713885267.415800, VS0, VE19 | null | null | en | og:image | https://wasp-lang.dev/docs/project/client-config | og:url | Client Config | Wasp | og:title | You can configure the client using the client field inside the app declaration: | og:description | Client Config | Wasp | null | Client Config
You can configure the client using the client field inside the app declaration:
JavaScript
TypeScript
main.wasp
app MyApp {
title: "My app",
// ...
client: {
rootComponent: import Root from "@src/Root.jsx",
setupFn: import mySetupFunction from "@src/myClientSetupCode.js"
}
}
Root Component
Wasp gives you the option to define a "wrapper" component for your React app.
It can be used for a variety of purposes, but the most common ones are:
Defining a common layout for your application.
Setting up various providers that your application needs.
Defining a Common Layout
Let's define a common layout for your application:
JavaScript
TypeScript
main.wasp
app MyApp {
title: "My app",
// ...
client: {
rootComponent: import Root from "@src/Root.jsx",
}
}
src/Root.jsx
export default function Root({ children }) {
return (
<div>
<header>
<h1>My App</h1>
</header>
{children}
<footer>
<p>My App footer</p>
</footer>
</div>
)
}
Setting up a Provider
This is how to set up various providers that your application needs:
JavaScript
TypeScript
main.wasp
app MyApp {
title: "My app",
// ...
client: {
rootComponent: import Root from "@src/Root.jsx",
}
}
src/Root.jsx
import store from './store'
import { Provider } from 'react-redux'
export default function Root({ children }) {
return <Provider store={store}>{children}</Provider>
}
As long as you render the children, you can do whatever you want in your root component.
Read more about the root component in the API Reference.
Setup Function
setupFn declares a function that Wasp executes on the client before everything else.
Running Some Code
We can run any code we want in the setup function.
For example, here's a setup function that logs a message every hour:
JavaScript
TypeScript
src/myClientSetupCode.js
export default async function mySetupFunction() {
let count = 1
setInterval(
() => console.log(`You have been online for ${count++} hours.`),
1000 * 60 * 60
)
}
Overriding Default Behaviour for Queries
info
You can change the options for a single Query using the options object, as described here.
Wasp's useQuery hook uses react-query's useQuery hook under the hood. Since react-query comes configured with aggressive but sane default options, you most likely won't have to change those defaults for all Queries.
If you do need to change the global defaults, you can do so inside the client setup function.
Wasp exposes a configureQueryClient hook that lets you configure react-query's QueryClient object:
JavaScript
TypeScript
src/myClientSetupCode.js
import { configureQueryClient } from 'wasp/client/operations'
export default async function mySetupFunction() {
// ... some setup
configureQueryClient({
defaultOptions: {
queries: {
staleTime: Infinity,
},
},
})
// ... some more setup
}
Make sure to pass in an object expected by the QueryClient's constructor, as explained in react-query's docs.
Read more about the setup function in the API Reference.
Base Directory
If you need to serve the client from a subdirectory, you can use the baseDir option:
main.wasp
app MyApp {
title: "My app",
// ...
client: {
baseDir: "/my-app",
}
}
This means that if you serve your app from https://example.com/my-app, the router will work correctly, and all the assets will be served from https://example.com/my-app.
Setting the correct env variable
If you set the baseDir option, make sure that the WASP_WEB_CLIENT_URL env variable also includes that base directory.
For example, if you are serving your app from https://example.com/my-app, the WASP_WEB_CLIENT_URL should be also set to https://example.com/my-app, and not just https://example.com.
API Reference
JavaScript
TypeScript
main.wasp
app MyApp {
title: "My app",
// ...
client: {
rootComponent: import Root from "@src/Root.jsx",
setupFn: import mySetupFunction from "@src/myClientSetupCode.js"
}
}
Client has the following options:
rootComponent: ExtImport
rootComponent defines the root component of your client application. It is expected to be a React component, and Wasp will use it to wrap your entire app. It must render its children, which are the actual pages of your application.
Here's an example of a root component that both sets up a provider and renders a custom layout:
JavaScript
TypeScript
src/Root.jsx
import store from './store'
import { Provider } from 'react-redux'
export default function Root({ children }) {
return (
<Provider store={store}>
<Layout>{children}</Layout>
</Provider>
)
}
function Layout({ children }) {
return (
<div>
<header>
<h1>My App</h1>
</header>
{children}
<footer>
<p>My App footer</p>
</footer>
</div>
)
}
setupFn: ExtImport
You can use this function to perform any custom setup (e.g., setting up client-side periodic jobs).
JavaScript
TypeScript
src/myClientSetupCode.js
export default async function mySetupFunction() {
// Run some code
}
baseDir: String
If you need to serve the client from a subdirectory, you can use the baseDir option.
If you set baseDir to /my-app for example, that will make Wasp set the basename prop of the Router to /my-app. It will also set the base option of the Vite config to /my-app.
This means that if you serve your app from https://example.com/my-app, the router will work correctly, and all the assets will be served from https://example.com/my-app.
Setting the correct env variable
If you set the baseDir option, make sure that the WASP_WEB_CLIENT_URL env variable also includes that base directory.
For example, if you are serving your app from https://example.com/my-app, the WASP_WEB_CLIENT_URL should be also set to https://example.com/my-app, and not just https://example.com. | https://wasp-lang.dev/docs/project/client-config |
|
1 | null | 2024-04-23T15:14:34.409Z | https://wasp-lang.dev/docs/project/static-assets | https://wasp-lang.dev/docs | http | ## Static Asset Handling
## Importing an Asset as URL[](#importing-an-asset-as-url "Direct link to Importing an Asset as URL")
Importing a static asset (e.g. an image) will return its URL. For example:
* JavaScript
* TypeScript
src/App.jsx
```
import imgUrl from './img.png'function App() { return <img src={imgUrl} alt="img" />}
```
For example, `imgUrl` will be `/img.png` during development, and become `/assets/img.2d8efhg.png` in the production build.
This is what you want to use most of the time, as it ensures that the asset file exists and is included in the bundle.
We are using Vite under the hood, read more about importing static assets in Vite's [docs](https://vitejs.dev/guide/assets.html#importing-asset-as-url).
## The `public` Directory[](#the-public-directory "Direct link to the-public-directory")
If you have assets that are:
* Never referenced in source code (e.g. robots.txt)
* Must retain the exact same file name (without hashing)
* ...or you simply don't want to have to import an asset first just to get its URL
Then you can place the asset in the `public` directory at the root of your project:
```
.└── public ├── favicon.ico └── robots.txt
```
Assets in this directory will be served at root path `/` during development and copied to the root of the dist directory as-is.
For example, if you have a file `favicon.ico` in the `public` directory, and your app is hosted at `https://myapp.com`, it will be made available at `https://myapp.com/favicon.ico`.
Usage in client code
Note that:
* You should always reference public assets using root absolute path
* for example, `public/icon.png` should be referenced in source code as `/icon.png`.
* Assets in the `public` directory **cannot be imported** from . | null | https://wasp-lang.dev/docs/project/static-assets | Importing an Asset as URL | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecdd4ac2707bd-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:34 GMT | Tue, 23 Apr 2024 15:24:34 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=7B7CJbticLe%2FJnzWcBMGsAJvCPWKny3iy%2FT9i0K0cdMcpRApWyn6yCojEfYvJ03ESv6l3dszIqy1rrJugUdmjXyU6G2Q5IU6kulJdcnMnH14KumQKmNw4P%2FfjpeKpeuF"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 160e8adc84c6c6f1a131b1307840dbe25b978a52 | null | 60EE:7E33C:3B77751:4658547:6627D059 | null | MISS | cache-iad-kiad7000079-IAD | S1713885274.371037,VS0,VE16 | null | null | en | og:image | https://wasp-lang.dev/docs/project/static-assets | og:url | Static Asset Handling | Wasp | og:title | Importing an Asset as URL | og:description | Static Asset Handling | Wasp | null | Static Asset Handling
Importing an Asset as URL
Importing a static asset (e.g. an image) will return its URL. For example:
JavaScript
TypeScript
src/App.jsx
import imgUrl from './img.png'
function App() {
return <img src={imgUrl} alt="img" />
}
For example, imgUrl will be /img.png during development, and become /assets/img.2d8efhg.png in the production build.
This is what you want to use most of the time, as it ensures that the asset file exists and is included in the bundle.
We are using Vite under the hood, read more about importing static assets in Vite's docs.
The public Directory
If you have assets that are:
Never referenced in source code (e.g. robots.txt)
Must retain the exact same file name (without hashing)
...or you simply don't want to have to import an asset first just to get its URL
Then you can place the asset in the public directory at the root of your project:
.
└── public
├── favicon.ico
└── robots.txt
Assets in this directory will be served at root path / during development and copied to the root of the dist directory as-is.
For example, if you have a file favicon.ico in the public directory, and your app is hosted at https://myapp.com, it will be made available at https://myapp.com/favicon.ico.
Usage in client code
Note that:
You should always reference public assets using root absolute path
for example, public/icon.png should be referenced in source code as /icon.png.
Assets in the public directory cannot be imported from . | https://wasp-lang.dev/docs/project/static-assets |
|
1 | null | 2024-04-23T15:14:37.434Z | https://wasp-lang.dev/docs/project/env-vars | https://wasp-lang.dev/docs | http | ## Env Variables
**Environment variables** are used to configure projects based on the context in which they run. This allows them to exhibit different behaviors in different environments, such as development, staging, or production.
For instance, _during development_, you may want your project to connect to a local development database running on your machine, but _in production_, you may prefer it to connect to the production database. Similarly, in development, you may want to use a test Stripe account, while in production, your app should use a real Stripe account.
While some env vars are required by Wasp, such as the database connection or secrets for social auth, you can also define your env vars for any other useful purposes.
In Wasp, you can use environment variables in both the client and the server code.
## Client Env Vars[](#client-env-vars "Direct link to Client Env Vars")
Client environment variables are embedded into the client code during the build and shipping process, making them public and readable by anyone. Therefore, you should **never store secrets in them** (such as secret API keys).
To enable Wasp to pick them up, client environment variables must be prefixed with `REACT_APP_`, for example: `REACT_APP_SOME_VAR_NAME=...`.
You can read them from the client code like this:
* JavaScript
* TypeScript
src/App.js
```
console.log(import.meta.env.REACT_APP_SOME_VAR_NAME)
```
Check below on how to define them.
## Server Env Vars[](#server-env-vars "Direct link to Server Env Vars")
In server environment variables, you can store secret values (e.g. secret API keys) since are not publicly readable. You can define them without any special prefix, such as `SOME_VAR_NAME=...`.
You can read them in the server code like this:
* JavaScript
* TypeScript
```
console.log(process.env.SOME_VAR_NAME)
```
Check below on how to define them.
## Defining Env Vars in Development[](#defining-env-vars-in-development "Direct link to Defining Env Vars in Development")
During development, there are two ways to provide env vars to your Wasp project:
1. Using `.env` files. **(recommended)**
2. Using shell. (useful for overrides)
### 1\. Using .env (dotenv) Files[](#1-using-env-dotenv-files "Direct link to 1. Using .env (dotenv) Files")

This is the recommended method for providing env vars to your Wasp project during development.
In the root of your Wasp project you can create two distinct files:
* `.env.server` for env vars that will be provided to the server.
Variables are defined in these files in the form of `NAME=VALUE`, for example:
.env.server
```
DATABASE_URL=postgresql://localhost:5432SOME_VAR_NAME=somevalue
```
* `.env.client` for env vars that will be provided to the client.
Variables are defined in these files in the form of `NAME=VALUE`, for example:
.env.client
```
REACT_APP_SOME_VAR_NAME=somevalue
```
These files should not be committed to version control, and they are already ignored by default in the `.gitignore` file that comes with Wasp.
### 2\. Using Shell[](#2-using-shell "Direct link to 2. Using Shell")
If you set environment variables in the shell where you run your Wasp commands (e.g., `wasp start`), Wasp will recognize them.
You can set environment variables in the `.profile` or a similar file, or by defining them at the start of a command:
```
SOME_VAR_NAME=SOMEVALUE wasp start
```
This is not specific to Wasp and is simply how environment variables can be set in the shell.
Defining environment variables in this way can be cumbersome even for a single project and even more challenging to manage if you have multiple Wasp projects. Therefore, we do not recommend this as a default method for providing environment variables to Wasp projects. However, it can be useful for occasionally **overriding** specific environment variables because environment variables set this way **take precedence over those defined in `.env` files**.
## Defining Env Vars in Production[](#defining-env-vars-in-production "Direct link to Defining Env Vars in Production")
While in development, we had the option of using `.env` files which made it easy to define and manage env vars. However, in production, we need to provide env vars differently.

### Client Env Vars[](#client-env-vars-1 "Direct link to Client Env Vars")
Client env vars are embedded into the client code during the build and shipping process, making them public and readable by anyone. Therefore, you should **never store secrets in them** (such as secret API keys).
You should provide them to the build command, for example:
```
REACT_APP_SOME_VAR_NAME=somevalue npm run build
```
How it works
What happens behind the scenes is that Wasp will replace all occurrences of `import.meta.env.REACT_APP_SOME_VAR_NAME` with the value you provided. This is done during the build process, so the value is embedded into the client code.
Read more about it in Vite's [docs](https://vitejs.dev/guide/env-and-mode.html#production-replacement).
### Server Env Vars[](#server-env-vars-1 "Direct link to Server Env Vars")
The way you provide env vars to your Wasp project in production depends on where you deploy it. For example, if you deploy your project to [Fly](https://fly.io/), you can define them using the `flyctl` CLI tool:
```
flyctl secrets set SOME_VAR_NAME=somevalue
```
You can read a lot more details in the [deployment section](https://wasp-lang.dev/docs/advanced/deployment/manually) of the docs. We go into detail on how to define env vars for each deployment option. | null | https://wasp-lang.dev/docs/project/env-vars | Environment variables are used to configure projects based on the context in which they run. This allows them to exhibit different behaviors in different environments, such as development, staging, or production. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecde78b4113bb-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:37 GMT | Tue, 23 Apr 2024 15:24:37 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=QWUTdYuVIiAWazcDON%2F7OOFz7YN6gZ%2B%2Birg6dJIUZf5WpYx4BcZZmSaQE%2BrPrQWyBB6orTVVGj1pcR2GPNqTF%2BWUbWlgws%2BcnPpUYcm%2BJ0aa7yJruHYuLD4PyosFBUV%2B"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | a753d98a7d05691a92f5af8327088988735dacc9 | null | 33BE:83772:3992F16:44AFF1F:6627D05D | null | MISS | cache-iad-kiad7000149-IAD | S1713885277.400342,VS0,VE12 | null | null | en | og:image | https://wasp-lang.dev/docs/project/env-vars | og:url | Env Variables | Wasp | og:title | Environment variables are used to configure projects based on the context in which they run. This allows them to exhibit different behaviors in different environments, such as development, staging, or production. | og:description | Env Variables | Wasp | null | Env Variables
Environment variables are used to configure projects based on the context in which they run. This allows them to exhibit different behaviors in different environments, such as development, staging, or production.
For instance, during development, you may want your project to connect to a local development database running on your machine, but in production, you may prefer it to connect to the production database. Similarly, in development, you may want to use a test Stripe account, while in production, your app should use a real Stripe account.
While some env vars are required by Wasp, such as the database connection or secrets for social auth, you can also define your env vars for any other useful purposes.
In Wasp, you can use environment variables in both the client and the server code.
Client Env Vars
Client environment variables are embedded into the client code during the build and shipping process, making them public and readable by anyone. Therefore, you should never store secrets in them (such as secret API keys).
To enable Wasp to pick them up, client environment variables must be prefixed with REACT_APP_, for example: REACT_APP_SOME_VAR_NAME=....
You can read them from the client code like this:
JavaScript
TypeScript
src/App.js
console.log(import.meta.env.REACT_APP_SOME_VAR_NAME)
Check below on how to define them.
Server Env Vars
In server environment variables, you can store secret values (e.g. secret API keys) since are not publicly readable. You can define them without any special prefix, such as SOME_VAR_NAME=....
You can read them in the server code like this:
JavaScript
TypeScript
console.log(process.env.SOME_VAR_NAME)
Check below on how to define them.
Defining Env Vars in Development
During development, there are two ways to provide env vars to your Wasp project:
Using .env files. (recommended)
Using shell. (useful for overrides)
1. Using .env (dotenv) Files
This is the recommended method for providing env vars to your Wasp project during development.
In the root of your Wasp project you can create two distinct files:
.env.server for env vars that will be provided to the server.
Variables are defined in these files in the form of NAME=VALUE, for example:
.env.server
DATABASE_URL=postgresql://localhost:5432
SOME_VAR_NAME=somevalue
.env.client for env vars that will be provided to the client.
Variables are defined in these files in the form of NAME=VALUE, for example:
.env.client
REACT_APP_SOME_VAR_NAME=somevalue
These files should not be committed to version control, and they are already ignored by default in the .gitignore file that comes with Wasp.
2. Using Shell
If you set environment variables in the shell where you run your Wasp commands (e.g., wasp start), Wasp will recognize them.
You can set environment variables in the .profile or a similar file, or by defining them at the start of a command:
SOME_VAR_NAME=SOMEVALUE wasp start
This is not specific to Wasp and is simply how environment variables can be set in the shell.
Defining environment variables in this way can be cumbersome even for a single project and even more challenging to manage if you have multiple Wasp projects. Therefore, we do not recommend this as a default method for providing environment variables to Wasp projects. However, it can be useful for occasionally overriding specific environment variables because environment variables set this way take precedence over those defined in .env files.
Defining Env Vars in Production
While in development, we had the option of using .env files which made it easy to define and manage env vars. However, in production, we need to provide env vars differently.
Client Env Vars
Client env vars are embedded into the client code during the build and shipping process, making them public and readable by anyone. Therefore, you should never store secrets in them (such as secret API keys).
You should provide them to the build command, for example:
REACT_APP_SOME_VAR_NAME=somevalue npm run build
How it works
What happens behind the scenes is that Wasp will replace all occurrences of import.meta.env.REACT_APP_SOME_VAR_NAME with the value you provided. This is done during the build process, so the value is embedded into the client code.
Read more about it in Vite's docs.
Server Env Vars
The way you provide env vars to your Wasp project in production depends on where you deploy it. For example, if you deploy your project to Fly, you can define them using the flyctl CLI tool:
flyctl secrets set SOME_VAR_NAME=somevalue
You can read a lot more details in the deployment section of the docs. We go into detail on how to define env vars for each deployment option. | https://wasp-lang.dev/docs/project/env-vars |
|
1 | null | 2024-04-23T15:14:43.126Z | https://wasp-lang.dev/docs/project/dependencies | https://wasp-lang.dev/docs | http | ## Dependencies
In a Wasp project, dependencies are defined in a standard way for JavaScript projects: using the [package.json](https://docs.npmjs.com/cli/configuring-npm/package-json) file, located at the root of your project. You can list your dependencies under the `dependencies` or `devDependencies` fields.
### Adding a New Dependency[](#adding-a-new-dependency "Direct link to Adding a New Dependency")
To add a new package, like `date-fns` (a great date handling library), you use `npm`:
This command will add the package in the `dependencies` section of your `package.json` file.
You will notice that there are some other packages in the `dependencies` section, like `react` and `wasp`. These are the packages that Wasp uses internally, and you should not modify or remove them.
### Using Packages that are Already Used by Wasp Internally[](#using-packages-that-are-already-used-by-wasp-internally "Direct link to Using Packages that are Already Used by Wasp Internally")
In the current version of Wasp, if Wasp is already internally using a certain dependency (e.g. React) with a certain version specified, you are not allowed to define that same npm dependency yourself while specifying _a different version_.
If you do that, you will get an error message telling you which exact version you have to use for that dependency. This means Wasp _dictates exact versions of certain packages_, so for example you can't choose the version of React you want to use.
note
We are currently working on a restructuring that will solve this and some other quirks: check [issue #734](https://github.com/wasp-lang/wasp/issues/734) to follow our progress. | null | https://wasp-lang.dev/docs/project/dependencies | In a Wasp project, dependencies are defined in a standard way for JavaScript projects: using the package.json file, located at the root of your project. You can list your dependencies under the dependencies or devDependencies fields. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece0b4e952003-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:43 GMT | Tue, 23 Apr 2024 15:12:13 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=XrXZikfs0i7zhomKfdeNH0%2BiuSaNOBYrBILXqGJKVXYOujd07FVHOO8GDAFayeYdnIOqikF%2F3jepYh81u2YAuHGMN00KIQ71Kr5ljQG5OMp1Evt2OFCerR2qukxk8Blv"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | HIT | 0 | 00494c37f828d044ce00b59f5f0cf86c624e4947 | null | 39EA:111A3A:3AC45EE:459FE1B:6627CD75 | null | MISS | cache-iad-kiad7000025-IAD | S1713885283.104369,VS0,VE6 | null | null | en | og:image | https://wasp-lang.dev/docs/project/dependencies | og:url | Dependencies | Wasp | og:title | In a Wasp project, dependencies are defined in a standard way for JavaScript projects: using the package.json file, located at the root of your project. You can list your dependencies under the dependencies or devDependencies fields. | og:description | Dependencies | Wasp | null | Dependencies
In a Wasp project, dependencies are defined in a standard way for JavaScript projects: using the package.json file, located at the root of your project. You can list your dependencies under the dependencies or devDependencies fields.
Adding a New Dependency
To add a new package, like date-fns (a great date handling library), you use npm:
This command will add the package in the dependencies section of your package.json file.
You will notice that there are some other packages in the dependencies section, like react and wasp. These are the packages that Wasp uses internally, and you should not modify or remove them.
Using Packages that are Already Used by Wasp Internally
In the current version of Wasp, if Wasp is already internally using a certain dependency (e.g. React) with a certain version specified, you are not allowed to define that same npm dependency yourself while specifying a different version.
If you do that, you will get an error message telling you which exact version you have to use for that dependency. This means Wasp dictates exact versions of certain packages, so for example you can't choose the version of React you want to use.
note
We are currently working on a restructuring that will solve this and some other quirks: check issue #734 to follow our progress. | https://wasp-lang.dev/docs/project/dependencies |
|
1 | null | 2024-04-23T15:14:43.153Z | https://wasp-lang.dev/docs/project/css-frameworks | https://wasp-lang.dev/docs | http | ## CSS Frameworks
## Tailwind[](#tailwind "Direct link to Tailwind")
To enable support for Tailwind in your project, you need to add two config files — [`tailwind.config.cjs`](https://tailwindcss.com/docs/configuration#configuration-options) and `postcss.config.cjs` — to the root directory.
With these files present, Wasp installs the necessary dependencies and copies your configuration to the generated project. You can then use [Tailwind CSS directives](https://tailwindcss.com/docs/functions-and-directives#directives) in your CSS and Tailwind classes on your React components.
tree .
```
.├── main.wasp├── package.json├── src│ ├── Main.css│ ├── MainPage.jsx│ ├── vite-env.d.ts│ └── waspLogo.png├── public├── tsconfig.json├── vite.config.ts├── postcss.config.cjs└── tailwind.config.cjs
```
Tailwind not working?
If you can not use Tailwind after adding the required config files, make sure to restart `wasp start`. This is sometimes needed to ensure that Wasp picks up the changes and enables Tailwind integration.
### Enabling Tailwind Step-by-Step[](#enabling-tailwind-step-by-step "Direct link to Enabling Tailwind Step-by-Step")
caution
Make sure to use the `.cjs` extension for these config files, if you name them with a `.js` extension, Wasp will not detect them.
1. Add `./tailwind.config.cjs`.
./tailwind.config.cjs
```
const { resolveProjectPath } = require('wasp/dev')/** @type {import('tailwindcss').Config} */module.exports = { content: [resolveProjectPath('./src/**/*.{js,jsx,ts,tsx}')], theme: { extend: {}, }, plugins: [],}
```
2. Add `./postcss.config.cjs`.
./postcss.config.cjs
```
module.exports = { plugins: { tailwindcss: {}, autoprefixer: {}, },}
```
3. Import Tailwind into your CSS file. For example, in a new project you might import Tailwind into `Main.css`.
./src/Main.css
```
@tailwind base;@tailwind components;@tailwind utilities;/* ... */
```
4. Start using Tailwind 🥳
./src/MainPage.jsx
```
// ...<h1 className="text-3xl font-bold underline"> Hello world!</h1>// ...
```
### Adding Tailwind Plugins[](#adding-tailwind-plugins "Direct link to Adding Tailwind Plugins")
To add Tailwind plugins, install them as npm development [dependencies](https://wasp-lang.dev/docs/project/dependencies) and add them to the plugins list in your `tailwind.config.cjs` file:
```
npm install -D @tailwindcss/formsnpm install -D @tailwindcss/typography
```
and also
./tailwind.config.cjs
```
/** @type {import('tailwindcss').Config} */module.exports = { // ... plugins: [ require('@tailwindcss/forms'), require('@tailwindcss/typography'), ], // ...}
``` | null | https://wasp-lang.dev/docs/project/css-frameworks | Tailwind | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece0b58879c58-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:43 GMT | Tue, 23 Apr 2024 15:24:43 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=R%2FyCBvQdMD6tlMNj2HcwpcFSlMpLJhodd2EYfRODVflqTNTJAa5g9NP30CskY2hvGuaPjWdLsdK91tvAosnVdBuYnK2pkWmSG4n%2FtyFreX%2F4TAA3nf5HUD1H1Q1CpSKT"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 940be603a20ee4247be42ef13d95471fc9a54f42 | null | 3EEA:EBB2F:38EF4B0:43CF750:6627D062 | null | MISS | cache-iad-kiad7000060-IAD | S1713885283.120702,VS0,VE11 | null | null | en | og:image | https://wasp-lang.dev/docs/project/css-frameworks | og:url | CSS Frameworks | Wasp | og:title | Tailwind | og:description | CSS Frameworks | Wasp | null | CSS Frameworks
Tailwind
To enable support for Tailwind in your project, you need to add two config files — tailwind.config.cjs and postcss.config.cjs — to the root directory.
With these files present, Wasp installs the necessary dependencies and copies your configuration to the generated project. You can then use Tailwind CSS directives in your CSS and Tailwind classes on your React components.
tree .
.
├── main.wasp
├── package.json
├── src
│ ├── Main.css
│ ├── MainPage.jsx
│ ├── vite-env.d.ts
│ └── waspLogo.png
├── public
├── tsconfig.json
├── vite.config.ts
├── postcss.config.cjs
└── tailwind.config.cjs
Tailwind not working?
If you can not use Tailwind after adding the required config files, make sure to restart wasp start. This is sometimes needed to ensure that Wasp picks up the changes and enables Tailwind integration.
Enabling Tailwind Step-by-Step
caution
Make sure to use the .cjs extension for these config files, if you name them with a .js extension, Wasp will not detect them.
Add ./tailwind.config.cjs.
./tailwind.config.cjs
const { resolveProjectPath } = require('wasp/dev')
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [resolveProjectPath('./src/**/*.{js,jsx,ts,tsx}')],
theme: {
extend: {},
},
plugins: [],
}
Add ./postcss.config.cjs.
./postcss.config.cjs
module.exports = {
plugins: {
tailwindcss: {},
autoprefixer: {},
},
}
Import Tailwind into your CSS file. For example, in a new project you might import Tailwind into Main.css.
./src/Main.css
@tailwind base;
@tailwind components;
@tailwind utilities;
/* ... */
Start using Tailwind 🥳
./src/MainPage.jsx
// ...
<h1 className="text-3xl font-bold underline">
Hello world!
</h1>
// ...
Adding Tailwind Plugins
To add Tailwind plugins, install them as npm development dependencies and add them to the plugins list in your tailwind.config.cjs file:
npm install -D @tailwindcss/forms
npm install -D @tailwindcss/typography
and also
./tailwind.config.cjs
/** @type {import('tailwindcss').Config} */
module.exports = {
// ...
plugins: [
require('@tailwindcss/forms'),
require('@tailwindcss/typography'),
],
// ...
} | https://wasp-lang.dev/docs/project/css-frameworks |
|
1 | 200 | 2024-04-23T15:14:40.605Z | https://wasp-lang.dev/docs/project/testing | https://wasp-lang.dev/docs | browser | Version: 0.13.0
## Testing
info
Wasp is in beta, so keep in mind there might be some kinks / bugs, and possibly some changes with testing support in the future. If you encounter any issues, reach out to us on [Discord](https://discord.gg/rzdnErX) and we will make sure to help you out!
## Testing Your React App[](#testing-your-react-app "Direct link to Testing Your React App")
Wasp enables you to quickly and easily write both unit tests and React component tests for your frontend code. Because Wasp uses [Vite](https://vitejs.dev/), we support testing web apps through [Vitest](https://vitest.dev/).
Included Libraries
### Writing Tests[](#writing-tests "Direct link to Writing Tests")
For Wasp to pick up your tests, they should be placed within the `src` directory and use an extension that matches [these glob patterns](https://vitest.dev/config#include). Some of the file names that Wasp will pick up as tests:
* `yourFile.test.ts`
* `YourComponent.spec.jsx`
Within test files, you can import your other source files as usual. For example, if you have a component `Counter.jsx`, you test it by creating a file in the same directory called `Counter.test.jsx` and import the component with `import Counter from './Counter'`.
### Running Tests[](#running-tests "Direct link to Running Tests")
Running `wasp test client` will start Vitest in watch mode and recompile your Wasp project when changes are made.
* If you want to see a real-time UI, pass `--ui` as an option.
* To run the tests just once, use `wasp test client run`.
All arguments after `wasp test client` are passed directly to the Vitest CLI, so check out [their documentation](https://vitest.dev/guide/cli.html) for all of the options.
Be Careful
You should not run `wasp test` while `wasp start` is running. Both will try to compile your project to `.wasp/out`.
### React Testing Helpers[](#react-testing-helpers "Direct link to React Testing Helpers")
Wasp provides several functions to help you write React tests:
* `renderInContext`: Takes a React component, wraps it inside a `QueryClientProvider` and `Router`, and renders it. This is the function you should use to render components in your React component tests.
```
import { renderInContext } from "wasp/client/test";renderInContext(<MainPage />);
```
* `mockServer`: Sets up the mock server and returns an object containing the `mockQuery` and `mockApi` utilities. This should be called outside of any test case, in each file that wants to use those helpers.
```
import { mockServer } from "wasp/client/test";const { mockQuery, mockApi } = mockServer();
```
* `mockQuery`: Takes a Wasp [query](https://wasp-lang.dev/docs/data-model/operations/queries) to mock and the JSON data it should return.
```
import { getTasks } from "wasp/client/operations";mockQuery(getTasks, []);
```
* Helpful when your component uses `useQuery`.
* Behind the scenes, Wasp uses [`msw`](https://npmjs.com/package/msw) to create a server request handle that responds with the specified data.
* Mock are cleared between each test.
* `mockApi`: Similar to `mockQuery`, but for [APIs](https://wasp-lang.dev/docs/advanced/apis). Instead of a Wasp query, it takes a route containing an HTTP method and a path.
```
import { HttpMethod } from "wasp/client";mockApi({ method: HttpMethod.Get, path: "/foor/bar" }, { res: "hello" });
```
## Testing Your Server-Side Code[](#testing-your-server-side-code "Direct link to Testing Your Server-Side Code")
Wasp currently does not provide a way to test your server-side code, but we will be adding support soon. You can track the progress at [this GitHub issue](https://github.com/wasp-lang/wasp/issues/110) and express your interest by commenting.
## Examples[](#examples "Direct link to Examples")
You can see some tests in a Wasp project [here](https://github.com/wasp-lang/wasp/blob/release/waspc/examples/todoApp/src/client/pages/auth/helpers.test.ts).
### Client Unit Tests[](#client-unit-tests "Direct link to Client Unit Tests")
* JavaScript
* TypeScript
src/helpers.js
```
export function areThereAnyTasks(tasks) { return tasks.length === 0;}
```
src/helpers.test.js
```
import { test, expect } from "vitest";import { areThereAnyTasks } from "./helpers";test("areThereAnyTasks", () => { expect(areThereAnyTasks([])).toBe(false);});
```
### React Component Tests[](#react-component-tests "Direct link to React Component Tests")
* JavaScript
* TypeScript
src/Todo.jsx
```
import { useQuery, getTasks } from "wasp/client/operations";const Todo = (_props) => { const { data: tasks } = useQuery(getTasks); return ( <ul> {tasks && tasks.map((task) => ( <li key={task.id}> <input type="checkbox" value={task.isDone} /> {task.description} </li> ))} </ul> );};
```
src/Todo.test.jsx
```
import { test, expect } from "vitest";import { screen } from "@testing-library/react";import { mockServer, renderInContext } from "wasp/client/test";import { getTasks } from "wasp/client/operations";import Todo from "./Todo";const { mockQuery } = mockServer();const mockTasks = [ { id: 1, description: "test todo 1", isDone: true, userId: 1, },];test("handles mock data", async () => { mockQuery(getTasks, mockTasks); renderInContext(<Todo />); await screen.findByText("test todo 1"); expect(screen.getByRole("checkbox")).toBeChecked(); screen.debug();});
```
### Testing With Mocked APIs[](#testing-with-mocked-apis "Direct link to Testing With Mocked APIs")
* JavaScript
* TypeScript
src/Todo.jsx
```
import { api } from "wasp/client/api";const Todo = (_props) => { const [tasks, setTasks] = useState([]); useEffect(() => { api .get("/tasks") .then((res) => res.json()) .then((tasks) => setTasks(tasks)) .catch((err) => window.alert(err)); }); return ( <ul> {tasks && tasks.map((task) => ( <li key={task.id}> <input type="checkbox" value={task.isDone} /> {task.description} </li> ))} </ul> );};
```
src/Todo.test.jsx
```
import { test, expect } from "vitest";import { screen } from "@testing-library/react";import { mockServer, renderInContext } from "wasp/client/test";import Todo from "./Todo";const { mockApi } = mockServer();const mockTasks = [ { id: 1, description: "test todo 1", isDone: true, userId: 1, },];test("handles mock data", async () => { mockApi("/tasks", { res: mockTasks }); renderInContext(<Todo />); await screen.findByText("test todo 1"); expect(screen.getByRole("checkbox")).toBeChecked(); screen.debug();});
``` | null | https://wasp-lang.dev/docs/project/testing | Wasp is in beta, so keep in mind there might be some kinks / bugs, and possibly some changes with testing support in the future. If you encounter any issues, reach out to us on Discord and we will make sure to help you out! | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecdeaadd24bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:37 GMT | Tue, 23 Apr 2024 15:24:37 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=iwlIKKFPfzpcPBtENTl2yxch1u22Ku2%2FB8FKKTaDEPFacqfp2q0xk49owi01XmwTY1bIXT2An0nLKmc4RG%2Fsd7Tlo%2FS9lZ93hYrO9f1F7doxBcq0zJM2xhANgqm6hfNe"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | b84cf3632b5cd5fd0e8e05c98158946193f9c21f | h2 | EF08:277B7:3B3419A:4650BB9:6627D05C | HIT | MISS | cache-nyc-kteb1890087-NYC | S1713885278.869506, VS0, VE16 | null | null | en | og:image | https://wasp-lang.dev/docs/project/testing | og:url | Testing | Wasp | og:title | Wasp is in beta, so keep in mind there might be some kinks / bugs, and possibly some changes with testing support in the future. If you encounter any issues, reach out to us on Discord and we will make sure to help you out! | og:description | Testing | Wasp | null | Version: 0.13.0
Testing
info
Wasp is in beta, so keep in mind there might be some kinks / bugs, and possibly some changes with testing support in the future. If you encounter any issues, reach out to us on Discord and we will make sure to help you out!
Testing Your React App
Wasp enables you to quickly and easily write both unit tests and React component tests for your frontend code. Because Wasp uses Vite, we support testing web apps through Vitest.
Included Libraries
Writing Tests
For Wasp to pick up your tests, they should be placed within the src directory and use an extension that matches these glob patterns. Some of the file names that Wasp will pick up as tests:
yourFile.test.ts
YourComponent.spec.jsx
Within test files, you can import your other source files as usual. For example, if you have a component Counter.jsx, you test it by creating a file in the same directory called Counter.test.jsx and import the component with import Counter from './Counter'.
Running Tests
Running wasp test client will start Vitest in watch mode and recompile your Wasp project when changes are made.
If you want to see a real-time UI, pass --ui as an option.
To run the tests just once, use wasp test client run.
All arguments after wasp test client are passed directly to the Vitest CLI, so check out their documentation for all of the options.
Be Careful
You should not run wasp test while wasp start is running. Both will try to compile your project to .wasp/out.
React Testing Helpers
Wasp provides several functions to help you write React tests:
renderInContext: Takes a React component, wraps it inside a QueryClientProvider and Router, and renders it. This is the function you should use to render components in your React component tests.
import { renderInContext } from "wasp/client/test";
renderInContext(<MainPage />);
mockServer: Sets up the mock server and returns an object containing the mockQuery and mockApi utilities. This should be called outside of any test case, in each file that wants to use those helpers.
import { mockServer } from "wasp/client/test";
const { mockQuery, mockApi } = mockServer();
mockQuery: Takes a Wasp query to mock and the JSON data it should return.
import { getTasks } from "wasp/client/operations";
mockQuery(getTasks, []);
Helpful when your component uses useQuery.
Behind the scenes, Wasp uses msw to create a server request handle that responds with the specified data.
Mock are cleared between each test.
mockApi: Similar to mockQuery, but for APIs. Instead of a Wasp query, it takes a route containing an HTTP method and a path.
import { HttpMethod } from "wasp/client";
mockApi({ method: HttpMethod.Get, path: "/foor/bar" }, { res: "hello" });
Testing Your Server-Side Code
Wasp currently does not provide a way to test your server-side code, but we will be adding support soon. You can track the progress at this GitHub issue and express your interest by commenting.
Examples
You can see some tests in a Wasp project here.
Client Unit Tests
JavaScript
TypeScript
src/helpers.js
export function areThereAnyTasks(tasks) {
return tasks.length === 0;
}
src/helpers.test.js
import { test, expect } from "vitest";
import { areThereAnyTasks } from "./helpers";
test("areThereAnyTasks", () => {
expect(areThereAnyTasks([])).toBe(false);
});
React Component Tests
JavaScript
TypeScript
src/Todo.jsx
import { useQuery, getTasks } from "wasp/client/operations";
const Todo = (_props) => {
const { data: tasks } = useQuery(getTasks);
return (
<ul>
{tasks &&
tasks.map((task) => (
<li key={task.id}>
<input type="checkbox" value={task.isDone} />
{task.description}
</li>
))}
</ul>
);
};
src/Todo.test.jsx
import { test, expect } from "vitest";
import { screen } from "@testing-library/react";
import { mockServer, renderInContext } from "wasp/client/test";
import { getTasks } from "wasp/client/operations";
import Todo from "./Todo";
const { mockQuery } = mockServer();
const mockTasks = [
{
id: 1,
description: "test todo 1",
isDone: true,
userId: 1,
},
];
test("handles mock data", async () => {
mockQuery(getTasks, mockTasks);
renderInContext(<Todo />);
await screen.findByText("test todo 1");
expect(screen.getByRole("checkbox")).toBeChecked();
screen.debug();
});
Testing With Mocked APIs
JavaScript
TypeScript
src/Todo.jsx
import { api } from "wasp/client/api";
const Todo = (_props) => {
const [tasks, setTasks] = useState([]);
useEffect(() => {
api
.get("/tasks")
.then((res) => res.json())
.then((tasks) => setTasks(tasks))
.catch((err) => window.alert(err));
});
return (
<ul>
{tasks &&
tasks.map((task) => (
<li key={task.id}>
<input type="checkbox" value={task.isDone} />
{task.description}
</li>
))}
</ul>
);
};
src/Todo.test.jsx
import { test, expect } from "vitest";
import { screen } from "@testing-library/react";
import { mockServer, renderInContext } from "wasp/client/test";
import Todo from "./Todo";
const { mockApi } = mockServer();
const mockTasks = [
{
id: 1,
description: "test todo 1",
isDone: true,
userId: 1,
},
];
test("handles mock data", async () => {
mockApi("/tasks", { res: mockTasks });
renderInContext(<Todo />);
await screen.findByText("test todo 1");
expect(screen.getByRole("checkbox")).toBeChecked();
screen.debug();
}); | https://wasp-lang.dev/docs/project/testing |
|
1 | null | 2024-04-23T15:14:45.456Z | https://wasp-lang.dev/docs/project/custom-vite-config | https://wasp-lang.dev/docs | http | Version: 0.13.0
## Custom Vite Config
Wasp uses [Vite](https://vitejs.dev/) to serve the client during development and bundling it for production. If you want to customize the Vite config, you can do that by editing the `vite.config.ts` file in your project root directory.
Wasp will use your config and **merge** it with the default Wasp's Vite config.
Vite config customization can be useful for things like:
* Adding custom Vite plugins.
* Customising the dev server.
* Customising the build process.
Be careful with making changes to the Vite config, as it can break the Wasp's client build process. Check out the default Vite config [here](https://github.com/wasp-lang/wasp/blob/release/waspc/data/Generator/templates/react-app/vite.config.ts) to see what you can change.
## Examples[](#examples "Direct link to Examples")
Below are some examples of how you can customize the Vite config.
### Changing the Dev Server Behaviour[](#changing-the-dev-server-behaviour "Direct link to Changing the Dev Server Behaviour")
If you want to stop Vite from opening the browser automatically when you run `wasp start`, you can do that by customizing the `open` option.
* JavaScript
* TypeScript
vite.config.js
```
export default { server: { open: false, },}
```
### Custom Dev Server Port[](#custom-dev-server-port "Direct link to Custom Dev Server Port")
You have access to all of the [Vite dev server options](https://vitejs.dev/config/server-options.html) in your custom Vite config. You can change the dev server port by setting the `port` option.
* JavaScript
* TypeScript
vite.config.js
```
export default { server: { port: 4000, },}
```
.env.server
```
WASP_WEB_CLIENT_URL=http://localhost:4000
```
Changing the dev server port
⚠️ Be careful when changing the dev server port, you'll need to update the `WASP_WEB_CLIENT_URL` env var in your `.env.server` file.
### Customising the Base Path[](#customising-the-base-path "Direct link to Customising the Base Path")
If you, for example, want to serve the client from a different path than `/`, you can do that by customizing the `base` option.
* JavaScript
* TypeScript
vite.config.js
```
export default { base: '/my-app/',}
``` | null | https://wasp-lang.dev/docs/project/custom-vite-config | Wasp uses Vite to serve the client during development and bundling it for production. If you want to customize the Vite config, you can do that by editing the vite.config.{js,ts} file in your project root directory. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece197acb080a-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:45 GMT | Tue, 23 Apr 2024 15:24:45 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=lRGp%2BQ135I5PO%2Fjm7%2FqVoXbW2kqQA57Lrz1NUc6e72WmWr91pW5AFH7bIkcEeLo1M7Osqewf1sfMQHIhT3SgS1gyELXfwT%2Fkd2pPkmP%2B7yxrjvvbZjyyXDskG3JddFgA"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | b21053cb877f2667e27988e8f4abc7160cc0732d | null | 64DC:3293B5:37E6F1E:42EC7CC:6627D065 | null | MISS | cache-iad-kiad7000044-IAD | S1713885285.374552,VS0,VE67 | null | null | en | og:image | https://wasp-lang.dev/docs/project/custom-vite-config | og:url | Custom Vite Config | Wasp | og:title | Wasp uses Vite to serve the client during development and bundling it for production. If you want to customize the Vite config, you can do that by editing the vite.config.{js,ts} file in your project root directory. | og:description | Custom Vite Config | Wasp | null | Version: 0.13.0
Custom Vite Config
Wasp uses Vite to serve the client during development and bundling it for production. If you want to customize the Vite config, you can do that by editing the vite.config.ts file in your project root directory.
Wasp will use your config and merge it with the default Wasp's Vite config.
Vite config customization can be useful for things like:
Adding custom Vite plugins.
Customising the dev server.
Customising the build process.
Be careful with making changes to the Vite config, as it can break the Wasp's client build process. Check out the default Vite config here to see what you can change.
Examples
Below are some examples of how you can customize the Vite config.
Changing the Dev Server Behaviour
If you want to stop Vite from opening the browser automatically when you run wasp start, you can do that by customizing the open option.
JavaScript
TypeScript
vite.config.js
export default {
server: {
open: false,
},
}
Custom Dev Server Port
You have access to all of the Vite dev server options in your custom Vite config. You can change the dev server port by setting the port option.
JavaScript
TypeScript
vite.config.js
export default {
server: {
port: 4000,
},
}
.env.server
WASP_WEB_CLIENT_URL=http://localhost:4000
Changing the dev server port
⚠️ Be careful when changing the dev server port, you'll need to update the WASP_WEB_CLIENT_URL env var in your .env.server file.
Customising the Base Path
If you, for example, want to serve the client from a different path than /, you can do that by customizing the base option.
JavaScript
TypeScript
vite.config.js
export default {
base: '/my-app/',
} | https://wasp-lang.dev/docs/project/custom-vite-config |
|
1 | null | 2024-04-23T15:14:45.425Z | https://wasp-lang.dev/docs/wasp-ai/creating-new-app | https://wasp-lang.dev/docs | http | Wasp comes with its own AI: Wasp AI, aka Mage (**M**agic web **A**pp **GE**nerator).
Wasp AI allows you to create a new Wasp app **from only a title and a short description** (using GPT in the background)!
There are two main ways to create a new Wasp app with Wasp AI:
1. Free, open-source online app [usemage.ai](https://usemage.ai/).
2. Running `wasp new` on your machine and picking AI generation. For this you need to provide your own OpenAI API keys, but it allows for more flexibility (choosing GPT models).
They both use the same logic in the background, so both approaches are equally "smart", the difference is just in the UI / settings.
info
Wasp AI is an experimental feature. Apps that Wasp AI generates can have mistakes (proportional to their complexity), but even then they can often serve as a great starting point (once you fix the mistakes) or an interesting way to explore how to implement stuff in Wasp.
## usemage.ai[](#usemageai "Direct link to usemage.ai")

1\. Describe your app 2. Pick the color 3. Generate your app 🚀
[Mage](https://usemage.ai/) is an open-source app with which you can create new Wasp apps from just a short title and description.
It is completely free for you - it uses our OpenAI API keys and we take on the costs.
Once you provide an app title, app description, and choose some basic settings, your new Wasp app will be created for you in a matter of minutes and you will be able to download it to your machine and keep working on it!
If you want to know more, check this [blog post](https://wasp-lang.dev/blog/2023/07/10/gpt-web-app-generator) for more details on how Mage works, or this [blog post](https://wasp-lang.dev/docs/wasp-ai/blog/2023/07/17/how-we-built-gpt-web-app-generator) for a high-level overview of how we implemented it.
## Wasp CLI[](#wasp-cli "Direct link to Wasp CLI")
You can create a new Wasp app using Wasp AI by running `wasp new` in your terminal and picking AI generation.
If you don't have them set yet, `wasp` will ask you to provide (via ENV vars) your OpenAI API keys (which it will use to query GPT).
Then, after providing a title and description for your Wasp app, the new app will be generated on your disk!
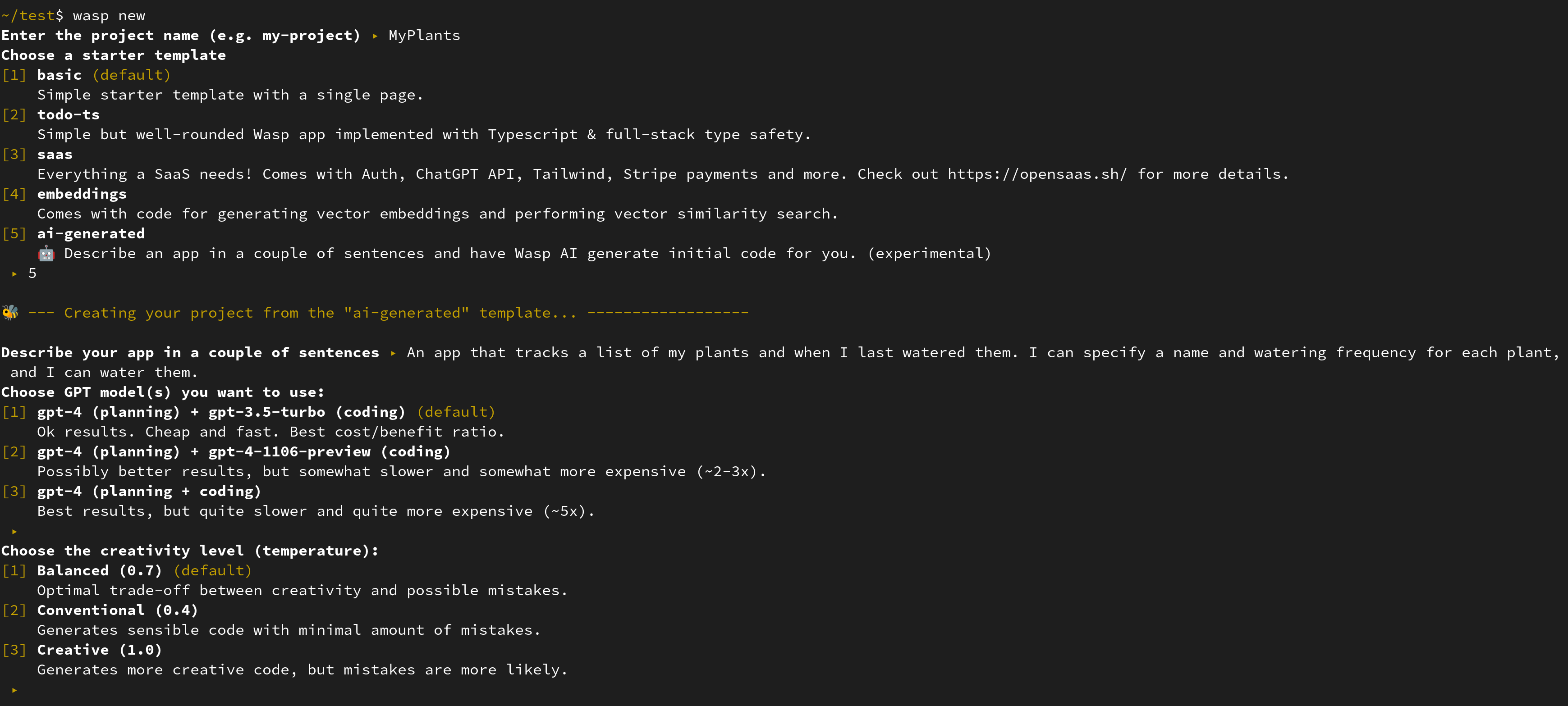 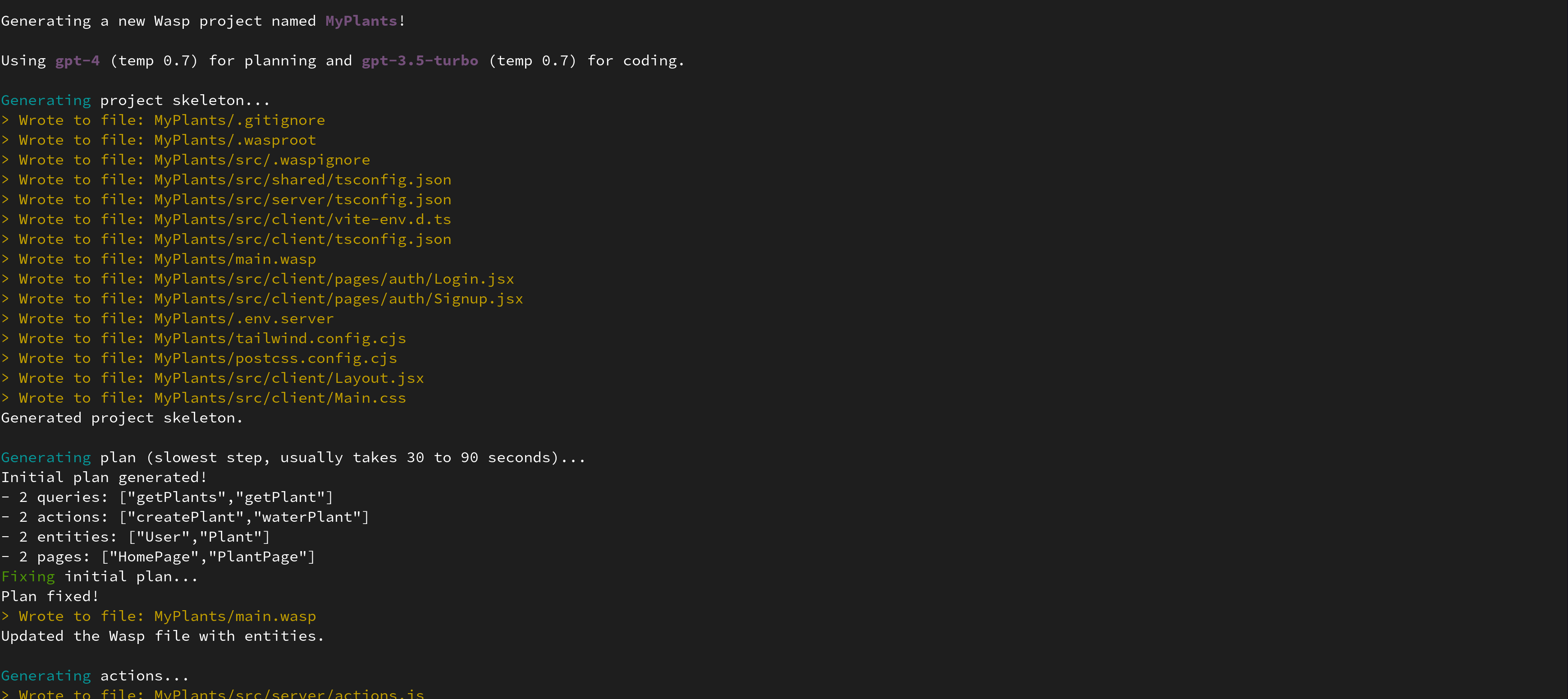 | null | https://wasp-lang.dev/docs/wasp-ai/creating-new-app | Wasp comes with its own AI: Wasp AI, aka Mage (Magic web App GEnerator). | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece199c5a398b-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:45 GMT | Tue, 23 Apr 2024 15:24:45 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=VomDLC0zpAwh%2FjU1aLKcsii6suFHjBduuZteCzuS%2FSDLEySXs%2FHWnnl93jAT5FrO3i2S5Ne3vKEthk%2Bbi5XtD2Yxq1W5jYWUc4QMbPM6n%2BaUmb3Bw5g7wCir%2BE%2FMFNON"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 52da165ee61cb795a4c04626cace8d0ae54fdd43 | null | 7852:3D5A5B:39A03CE:44A67A6:6627D065 | null | MISS | cache-iad-kiad7000073-IAD | S1713885285.392533,VS0,VE16 | null | null | en | og:image | https://wasp-lang.dev/docs/wasp-ai/creating-new-app | og:url | Creating New App with AI | Wasp | og:title | Wasp comes with its own AI: Wasp AI, aka Mage (Magic web App GEnerator). | og:description | Creating New App with AI | Wasp | null | Wasp comes with its own AI: Wasp AI, aka Mage (Magic web App GEnerator).
Wasp AI allows you to create a new Wasp app from only a title and a short description (using GPT in the background)!
There are two main ways to create a new Wasp app with Wasp AI:
Free, open-source online app usemage.ai.
Running wasp new on your machine and picking AI generation. For this you need to provide your own OpenAI API keys, but it allows for more flexibility (choosing GPT models).
They both use the same logic in the background, so both approaches are equally "smart", the difference is just in the UI / settings.
info
Wasp AI is an experimental feature. Apps that Wasp AI generates can have mistakes (proportional to their complexity), but even then they can often serve as a great starting point (once you fix the mistakes) or an interesting way to explore how to implement stuff in Wasp.
usemage.ai
1. Describe your app 2. Pick the color 3. Generate your app 🚀
Mage is an open-source app with which you can create new Wasp apps from just a short title and description.
It is completely free for you - it uses our OpenAI API keys and we take on the costs.
Once you provide an app title, app description, and choose some basic settings, your new Wasp app will be created for you in a matter of minutes and you will be able to download it to your machine and keep working on it!
If you want to know more, check this blog post for more details on how Mage works, or this blog post for a high-level overview of how we implemented it.
Wasp CLI
You can create a new Wasp app using Wasp AI by running wasp new in your terminal and picking AI generation.
If you don't have them set yet, wasp will ask you to provide (via ENV vars) your OpenAI API keys (which it will use to query GPT).
Then, after providing a title and description for your Wasp app, the new app will be generated on your disk! | https://wasp-lang.dev/docs/wasp-ai/creating-new-app |
|
1 | null | 2024-04-23T15:14:48.800Z | https://wasp-lang.dev/docs/wasp-ai/developing-existing-app | https://wasp-lang.dev/docs | http | Version: 0.13.0
While Wasp AI doesn't at the moment offer any additional help for developing your Wasp app with AI beyond initial generation, this is something we are exploring actively.
In the meantime, while waiting for Wasp AI to add support for this, we suggest checking out [aider](https://github.com/paul-gauthier/aider), which is an AI pair programming tool in your terminal. This is a third-party tool, not affiliated with Wasp in any way, but we and some of Wasp users have found that it can be helpful when working on Wasp apps. | null | https://wasp-lang.dev/docs/wasp-ai/developing-existing-app | While Wasp AI doesn't at the moment offer any additional help for developing your Wasp app with AI beyond initial generation, this is something we are exploring actively. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece2ebedb3b1a-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:48 GMT | Tue, 23 Apr 2024 15:24:48 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=vBacBDMkeVE%2FFXOx57lkbElreqFdItG6jxi0vmnyrtS%2BAstcZ%2FpKLuCGSDJsYQJYvyJAROkvEgGEYIyv6sPffV2j5B0TROXqAR8IMhWhHNDJhcJ%2B8nZ9SPtwxpOXIhxs"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 7b217813cbfc3924e4fb6b2b7251a932b8b822f6 | null | 96DE:35D1BB:3B391E9:465627E:6627D068 | null | MISS | cache-iad-kiad7000175-IAD | S1713885289.776890,VS0,VE12 | null | null | en | og:image | https://wasp-lang.dev/docs/wasp-ai/developing-existing-app | og:url | Developing Existing App with AI | Wasp | og:title | While Wasp AI doesn't at the moment offer any additional help for developing your Wasp app with AI beyond initial generation, this is something we are exploring actively. | og:description | Developing Existing App with AI | Wasp | null | Version: 0.13.0
While Wasp AI doesn't at the moment offer any additional help for developing your Wasp app with AI beyond initial generation, this is something we are exploring actively.
In the meantime, while waiting for Wasp AI to add support for this, we suggest checking out aider, which is an AI pair programming tool in your terminal. This is a third-party tool, not affiliated with Wasp in any way, but we and some of Wasp users have found that it can be helpful when working on Wasp apps. | https://wasp-lang.dev/docs/wasp-ai/developing-existing-app |
|
1 | null | 2024-04-23T15:14:50.217Z | https://wasp-lang.dev/docs/advanced/deployment/overview | https://wasp-lang.dev/docs | http | ## Overview
Wasp apps are full-stack apps that consist of:
* A Node.js server.
* A static client.
* A PostgreSQL database.
You can deploy each part **anywhere** where you can usually deploy Node.js apps or static apps. For example, you can deploy your client on [Netlify](https://www.netlify.com/), the server on [Fly.io](https://fly.io/), and the database on [Neon](https://neon.tech/).
To make deploying as smooth as possible, Wasp also offers a single-command deployment through the **Wasp CLI**.
Click on each deployment method for more details.
Regardless of how you choose to deploy your app (i.e., manually or using the Wasp CLI), you'll need to know about some common patterns covered below.
## Customizing the Dockerfile[](#customizing-the-dockerfile "Direct link to Customizing the Dockerfile")
By default, Wasp generates a multi-stage Dockerfile. This file is used to build and run a Docker image with the Wasp-generated server code. It also runs any pending migrations.
You can **add extra steps to this multi-stage `Dockerfile`** by creating your own `Dockerfile` in the project's root directory. If Wasp finds a Dockerfile in the project's root, it appends its contents at the _bottom_ of the default multi-stage Dockerfile.
Since the last definition in a Dockerfile wins, you can override or continue from any existing build stages. You can also choose not to use any of our build stages and have your own custom Dockerfile used as-is.
A few things to keep in mind:
* If you override an intermediate build stage, no later build stages will be used unless you reproduce them below.
* The generated Dockerfile's content is dynamic and depends on which features your app uses. The content can also change in future releases, so please verify it from time to time.
* Make sure to supply `ENTRYPOINT` in your final build stage. Your changes won't have any effect if you don't.
Read more in the official Docker docs on [multi-stage builds](https://docs.docker.com/build/building/multi-stage/).
To see what your project's (potentially combined) Dockerfile will look like, run:
Join our [Discord](https://discord.gg/rzdnErX) if you have any questions, or if you need more customization than this hook provides. | null | https://wasp-lang.dev/docs/advanced/deployment/overview | Wasp apps are full-stack apps that consist of: | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece3788ae818c-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:50 GMT | Tue, 23 Apr 2024 15:24:50 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=e6IuY0q%2F7iZJS8ugziYmPz4f3bUZfUgb2MEJU1DzBavrG8TeW1r88emAtYV%2BxtD2CiJBnJ8uoxnzhxlwWbiC2w4%2FDqUR6h7oJqjGl7O2MTU%2BUJGI6t4cJIvjOdl%2Fqn3I"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 990f3441a863c197b00d2812d004620a01e8f6b1 | null | D594:2C39F4:3B2D4A5:4649A16:6627D069 | HIT | MISS | cache-iad-kiad7000036-IAD | S1713885290.188822,VS0,VE13 | null | null | en | og:image | https://wasp-lang.dev/docs/advanced/deployment/overview | og:url | Overview | Wasp | og:title | Wasp apps are full-stack apps that consist of: | og:description | Overview | Wasp | null | Overview
Wasp apps are full-stack apps that consist of:
A Node.js server.
A static client.
A PostgreSQL database.
You can deploy each part anywhere where you can usually deploy Node.js apps or static apps. For example, you can deploy your client on Netlify, the server on Fly.io, and the database on Neon.
To make deploying as smooth as possible, Wasp also offers a single-command deployment through the Wasp CLI.
Click on each deployment method for more details.
Regardless of how you choose to deploy your app (i.e., manually or using the Wasp CLI), you'll need to know about some common patterns covered below.
Customizing the Dockerfile
By default, Wasp generates a multi-stage Dockerfile. This file is used to build and run a Docker image with the Wasp-generated server code. It also runs any pending migrations.
You can add extra steps to this multi-stage Dockerfile by creating your own Dockerfile in the project's root directory. If Wasp finds a Dockerfile in the project's root, it appends its contents at the bottom of the default multi-stage Dockerfile.
Since the last definition in a Dockerfile wins, you can override or continue from any existing build stages. You can also choose not to use any of our build stages and have your own custom Dockerfile used as-is.
A few things to keep in mind:
If you override an intermediate build stage, no later build stages will be used unless you reproduce them below.
The generated Dockerfile's content is dynamic and depends on which features your app uses. The content can also change in future releases, so please verify it from time to time.
Make sure to supply ENTRYPOINT in your final build stage. Your changes won't have any effect if you don't.
Read more in the official Docker docs on multi-stage builds.
To see what your project's (potentially combined) Dockerfile will look like, run:
Join our Discord if you have any questions, or if you need more customization than this hook provides. | https://wasp-lang.dev/docs/advanced/deployment/overview |
|
1 | null | 2024-04-23T15:14:50.249Z | https://wasp-lang.dev/docs/advanced/deployment/cli | https://wasp-lang.dev/docs | http | Wasp CLI can deploy your full-stack application with only a single command. The command automates the manual deployment process and is the recommended way of deploying Wasp apps.
## Supported Providers[](#supported-providers "Direct link to Supported Providers")
Wasp supports automated deployment to the following providers:
* [Fly.io](#flyio) - they offer 5$ free credit each month
* Railway (coming soon, track it here [#1157](https://github.com/wasp-lang/wasp/pull/1157))
## Fly.io[](#flyio "Direct link to Fly.io")
### Prerequisites[](#prerequisites "Direct link to Prerequisites")
Fly provides [free allowances](https://fly.io/docs/about/pricing/#plans) for up to 3 VMs (so deploying a Wasp app to a new account is free), but all plans require you to add your credit card information before you can proceed. If you don't, the deployment will fail.
You can add the required credit card information on the [account's billing page](https://fly.io/dashboard/personal/billing).
Fly.io CLI
You will need the [`flyctl` CLI](https://fly.io/docs/hands-on/install-flyctl/) installed on your machine before you can deploy to Fly.io.
### Deploying[](#deploying "Direct link to Deploying")
Using the Wasp CLI, you can easily deploy a new app to [Fly.io](https://fly.io/) with just a single command:
```
wasp deploy fly launch my-wasp-app mia
```
Specifying Org
If your account is a member of more than one organization on Fly.io, you will need to specify under which one you want to execute the command. To do that, provide an additional `--org <org-slug>` option. You can find out the names(slugs) of your organizations by running `fly orgs list`.
Please do not CTRL-C or exit your terminal while the commands are running.
Under the covers, this runs the equivalent of the following commands:
```
wasp deploy fly setup my-wasp-app miawasp deploy fly create-db miawasp deploy fly deploy
```
The commands above use the app basename `my-wasp-app` and deploy it to the _Miami, Florida (US) region_ (called `mia`). Read more about Fly.io regions [here](#flyio-regions).
Unique Name
Your app name must be unique across all of Fly or deployment will fail.
The basename is used to create all three app tiers, resulting in three separate apps in your Fly dashboard:
* `my-wasp-app-client`
* `my-wasp-app-server`
* `my-wasp-app-db`
You'll notice that Wasp creates two new files in your project root directory:
* `fly-server.toml`
* `fly-client.toml`
You should include these files in your version control so that you can deploy your app with a single command in the future.
### Using a Custom Domain For Your App[](#using-a-custom-domain-for-your-app "Direct link to Using a Custom Domain For Your App")
Setting up a custom domain is a three-step process:
1. You need to add your domain to your Fly client app. You can do this by running:
```
wasp deploy fly cmd --context client certs create mycoolapp.com
```
Use Your Domain
Make sure to replace `mycoolapp.com` with your domain in all of the commands mentioned in this section.
This command will output the instructions to add the DNS records to your domain. It will look something like this:
```
You can direct traffic to mycoolapp.com by:1: Adding an A record to your DNS service which reads A @ 66.241.1XX.154You can validate your ownership of mycoolapp.com by:2: Adding an AAAA record to your DNS service which reads: AAAA @ 2a09:82XX:1::1:ff40
```
2. You need to add the DNS records for your domain:
_This will depend on your domain provider, but it should be a matter of adding an A record for `@` and an AAAA record for `@` with the values provided by the previous command._
3. You need to set your domain as the `WASP_WEB_CLIENT_URL` environment variable for your server app:
```
wasp deploy fly cmd --context server secrets set WASP_WEB_CLIENT_URL=https://mycoolapp.com
```
We need to do this to keep our CORS configuration up to date.
That's it, your app should be available at `https://mycoolapp.com`! 🎉
## API Reference[](#api-reference "Direct link to API Reference")
### `launch`[](#launch "Direct link to launch")
`launch` is a convenience command that runs `setup`, `create-db`, and `deploy` in sequence.
```
wasp deploy fly launch <app-name> <region>
```
It accepts the following arguments:
* `<app-name>` - the name of your app required
* `<region>` - the region where your app will be deployed required
Read how to find the available regions [here](#flyio-regions).
It gives you the same result as running the following commands:
```
wasp deploy fly setup <app-name> <region>wasp deploy fly create-db <region>wasp deploy fly deploy
```
#### Environment Variables[](#environment-variables "Direct link to Environment Variables")
If you are deploying an app that requires any other environment variables (like social auth secrets), you can set them with the `--server-secret` option:
```
wasp deploy fly launch my-wasp-app mia --server-secret GOOGLE_CLIENT_ID=<...> --server-secret GOOGLE_CLIENT_SECRET=<...>
```
### `setup`[](#setup "Direct link to setup")
`setup` will create your client and server apps on Fly, and add some secrets, but does _not_ deploy them.
```
wasp deploy fly setup <app-name> <region>
```
It accepts the following arguments:
* `<app-name>` - the name of your app required
* `<region>` - the region where your app will be deployed required
Read how to find the available regions [here](#flyio-regions).
After running `setup`, Wasp creates two new files in your project root directory: `fly-server.toml` and `fly-client.toml`. You should include these files in your version control.
You **can edit the `fly-server.toml` and `fly-client.toml` files** to further configure your Fly deployments. Wasp will use the TOML files when you run `deploy`.
If you want to maintain multiple apps, you can add the `--fly-toml-dir <abs-path>` option to point to different directories, like "dev" or "staging".
Execute Only Once
You should only run `setup` once per app. If you run it multiple times, it will create unnecessary apps on Fly.
### `create-db`[](#create-db "Direct link to create-db")
`create-db` will create a new database for your app.
```
wasp deploy fly create-db <region>
```
It accepts the following arguments:
* `<region>` - the region where your app will be deployed required
Read how to find the available regions [here](#flyio-regions).
Execute Only Once
You should only run `create-db` once per app. If you run it multiple times, it will create multiple databases, but your app needs only one.
### `deploy`[](#deploy "Direct link to deploy")
`deploy` pushes your client and server live.
Run this command whenever you want to **update your deployed app** with the latest changes:
### `cmd`[](#cmd "Direct link to cmd")
If want to run arbitrary Fly commands (e.g. `flyctl secrets list` for your server app), here's how to do it:
```
wasp deploy fly cmd secrets list --context server
```
### Fly.io Regions[](#flyio-regions "Direct link to Fly.io Regions")
> Fly.io runs applications physically close to users: in datacenters around the world, on servers we run ourselves. You can currently deploy your apps in 34 regions, connected to a global Anycast network that makes sure your users hit our nearest server, whether they’re in Tokyo, São Paolo, or Frankfurt.
Read more on Fly regions [here](https://fly.io/docs/reference/regions/).
You can find the list of all available Fly regions by running:
#### Environment Variables[](#environment-variables-1 "Direct link to Environment Variables")
If you are deploying an app that requires any other environment variables (like social auth secrets), you can set them with the `secrets set` command:
```
wasp deploy fly cmd secrets set GOOGLE_CLIENT_ID=<...> GOOGLE_CLIENT_SECRET=<...> --context=server
```
### Multiple Fly Organizations[](#multiple-fly-organizations "Direct link to Multiple Fly Organizations")
If you have multiple organizations, you can specify a `--org` option. For example:
```
wasp deploy fly launch my-wasp-app mia --org hive
```
### Building Locally[](#building-locally "Direct link to Building Locally")
Fly.io offers support for both **locally** built Docker containers and **remotely** built ones. However, for simplicity and reproducibility, the CLI defaults to the use of a remote Fly.io builder.
If you want to build locally, supply the `--build-locally` option to `wasp deploy fly launch` or `wasp deploy fly deploy`. | null | https://wasp-lang.dev/docs/advanced/deployment/cli | Wasp CLI can deploy your full-stack application with only a single command. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece3779968f29-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:50 GMT | Tue, 23 Apr 2024 15:24:50 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=%2F1nxZjyfKzk5OsZdTa9uYIBgQT2vPjiMQ2Ri8czWANOd1avK0iBeHUXL8srp9gdlwqBc2KyjebCy3%2FXbvzxcUJsmIetLZqZnBg7htJqSFQJ8pA6IUKVTsS%2ByPRqvJ20o"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | f80db28346e54ec14997307db19a01737c84f471 | null | F3C2:3D5A5B:39A07B3:44A6C20:6627D069 | null | MISS | cache-iad-kiad7000087-IAD | S1713885290.207560,VS0,VE16 | null | null | en | og:image | https://wasp-lang.dev/docs/advanced/deployment/cli | og:url | Deploying with the Wasp CLI | Wasp | og:title | Wasp CLI can deploy your full-stack application with only a single command. | og:description | Deploying with the Wasp CLI | Wasp | null | Wasp CLI can deploy your full-stack application with only a single command. The command automates the manual deployment process and is the recommended way of deploying Wasp apps.
Supported Providers
Wasp supports automated deployment to the following providers:
Fly.io - they offer 5$ free credit each month
Railway (coming soon, track it here #1157)
Fly.io
Prerequisites
Fly provides free allowances for up to 3 VMs (so deploying a Wasp app to a new account is free), but all plans require you to add your credit card information before you can proceed. If you don't, the deployment will fail.
You can add the required credit card information on the account's billing page.
Fly.io CLI
You will need the flyctl CLI installed on your machine before you can deploy to Fly.io.
Deploying
Using the Wasp CLI, you can easily deploy a new app to Fly.io with just a single command:
wasp deploy fly launch my-wasp-app mia
Specifying Org
If your account is a member of more than one organization on Fly.io, you will need to specify under which one you want to execute the command. To do that, provide an additional --org <org-slug> option. You can find out the names(slugs) of your organizations by running fly orgs list.
Please do not CTRL-C or exit your terminal while the commands are running.
Under the covers, this runs the equivalent of the following commands:
wasp deploy fly setup my-wasp-app mia
wasp deploy fly create-db mia
wasp deploy fly deploy
The commands above use the app basename my-wasp-app and deploy it to the Miami, Florida (US) region (called mia). Read more about Fly.io regions here.
Unique Name
Your app name must be unique across all of Fly or deployment will fail.
The basename is used to create all three app tiers, resulting in three separate apps in your Fly dashboard:
my-wasp-app-client
my-wasp-app-server
my-wasp-app-db
You'll notice that Wasp creates two new files in your project root directory:
fly-server.toml
fly-client.toml
You should include these files in your version control so that you can deploy your app with a single command in the future.
Using a Custom Domain For Your App
Setting up a custom domain is a three-step process:
You need to add your domain to your Fly client app. You can do this by running:
wasp deploy fly cmd --context client certs create mycoolapp.com
Use Your Domain
Make sure to replace mycoolapp.com with your domain in all of the commands mentioned in this section.
This command will output the instructions to add the DNS records to your domain. It will look something like this:
You can direct traffic to mycoolapp.com by:
1: Adding an A record to your DNS service which reads
A @ 66.241.1XX.154
You can validate your ownership of mycoolapp.com by:
2: Adding an AAAA record to your DNS service which reads:
AAAA @ 2a09:82XX:1::1:ff40
You need to add the DNS records for your domain:
This will depend on your domain provider, but it should be a matter of adding an A record for @ and an AAAA record for @ with the values provided by the previous command.
You need to set your domain as the WASP_WEB_CLIENT_URL environment variable for your server app:
wasp deploy fly cmd --context server secrets set WASP_WEB_CLIENT_URL=https://mycoolapp.com
We need to do this to keep our CORS configuration up to date.
That's it, your app should be available at https://mycoolapp.com! 🎉
API Reference
launch
launch is a convenience command that runs setup, create-db, and deploy in sequence.
wasp deploy fly launch <app-name> <region>
It accepts the following arguments:
<app-name> - the name of your app required
<region> - the region where your app will be deployed required
Read how to find the available regions here.
It gives you the same result as running the following commands:
wasp deploy fly setup <app-name> <region>
wasp deploy fly create-db <region>
wasp deploy fly deploy
Environment Variables
If you are deploying an app that requires any other environment variables (like social auth secrets), you can set them with the --server-secret option:
wasp deploy fly launch my-wasp-app mia --server-secret GOOGLE_CLIENT_ID=<...> --server-secret GOOGLE_CLIENT_SECRET=<...>
setup
setup will create your client and server apps on Fly, and add some secrets, but does not deploy them.
wasp deploy fly setup <app-name> <region>
It accepts the following arguments:
<app-name> - the name of your app required
<region> - the region where your app will be deployed required
Read how to find the available regions here.
After running setup, Wasp creates two new files in your project root directory: fly-server.toml and fly-client.toml. You should include these files in your version control.
You can edit the fly-server.toml and fly-client.toml files to further configure your Fly deployments. Wasp will use the TOML files when you run deploy.
If you want to maintain multiple apps, you can add the --fly-toml-dir <abs-path> option to point to different directories, like "dev" or "staging".
Execute Only Once
You should only run setup once per app. If you run it multiple times, it will create unnecessary apps on Fly.
create-db
create-db will create a new database for your app.
wasp deploy fly create-db <region>
It accepts the following arguments:
<region> - the region where your app will be deployed required
Read how to find the available regions here.
Execute Only Once
You should only run create-db once per app. If you run it multiple times, it will create multiple databases, but your app needs only one.
deploy
deploy pushes your client and server live.
Run this command whenever you want to update your deployed app with the latest changes:
cmd
If want to run arbitrary Fly commands (e.g. flyctl secrets list for your server app), here's how to do it:
wasp deploy fly cmd secrets list --context server
Fly.io Regions
Fly.io runs applications physically close to users: in datacenters around the world, on servers we run ourselves. You can currently deploy your apps in 34 regions, connected to a global Anycast network that makes sure your users hit our nearest server, whether they’re in Tokyo, São Paolo, or Frankfurt.
Read more on Fly regions here.
You can find the list of all available Fly regions by running:
Environment Variables
If you are deploying an app that requires any other environment variables (like social auth secrets), you can set them with the secrets set command:
wasp deploy fly cmd secrets set GOOGLE_CLIENT_ID=<...> GOOGLE_CLIENT_SECRET=<...> --context=server
Multiple Fly Organizations
If you have multiple organizations, you can specify a --org option. For example:
wasp deploy fly launch my-wasp-app mia --org hive
Building Locally
Fly.io offers support for both locally built Docker containers and remotely built ones. However, for simplicity and reproducibility, the CLI defaults to the use of a remote Fly.io builder.
If you want to build locally, supply the --build-locally option to wasp deploy fly launch or wasp deploy fly deploy. | https://wasp-lang.dev/docs/advanced/deployment/cli |
|
1 | null | 2024-04-23T15:14:50.622Z | https://wasp-lang.dev/docs/advanced/deployment/manually | https://wasp-lang.dev/docs | http | ## Deploying Manually
This document explains how to build and prepare your Wasp app for deployment. You can then deploy the built Wasp app wherever and however you want, as long as your provider/server supports Wasp's build format.
After going through the general steps that apply to all deployments, you can follow step-by-step guides for deploying your Wasp app to the most popular providers:
* [Fly.io](#flyio)
* [Netlify](#netlify)
* [Railway](#railway)
* [Heroku](#heroku)
No worries, you can still deploy your app if your desired provider isn't on the list - it just means we don't yet have a step-by-step guide for you to follow. Feel free to [open a PR](https://github.com/wasp-lang/wasp/edit/release/web/docs/advanced/deployment/manually.md) if you'd like to write one yourself :)
## Deploying a Wasp App[](#deploying-a-wasp-app "Direct link to Deploying a Wasp App")
Deploying a Wasp app comes down to the following:
1. Generating deployable code.
2. Deploying the API server (backend).
3. Deploying the web client (frontend).
4. Deploying a PostgreSQL database and keeping it running.
Let's go through each of these steps.
### 1\. Generating Deployable Code[](#1-generating-deployable-code "Direct link to 1. Generating Deployable Code")
Running the command `wasp build` generates deployable code for the whole app in the `.wasp/build/` directory.
PostgreSQL in production
You won't be able to build the app if you are using SQLite as a database (which is the default database). You'll have to [switch to PostgreSQL](https://wasp-lang.dev/docs/data-model/backends#migrating-from-sqlite-to-postgresql) before deploying to production.
### 2\. Deploying the API Server (backend)[](#2-deploying-the-api-server-backend "Direct link to 2. Deploying the API Server (backend)")
There's a Dockerfile that defines an image for building the server in the `.wasp/build` directory.
To run the server in production, deploy this Docker image to a hosting provider and ensure the required environment variables on the provider are correctly set up (the mechanism of setting these up is specific per provider). All necessary environment variables are listed in the next section.
#### Environment Variables[](#environment-variables "Direct link to Environment Variables")
Here are the environment variables your server will be looking for:
* `DATABASE_URL` required
The URL of the PostgreSQL database you want your app to use (e.g., `postgresql://mydbuser:mypass@localhost:5432/nameofmydb`).
* `WASP_WEB_CLIENT_URL` required
The URL where you plan to deploy your frontend app is running (e.g., `https://<app-name>.netlify.app`). The server needs to know about it to properly configure Same-Origin Policy (CORS) headers.
* `WASP_SERVER_URL` required
The URL where the server is running (e.g., `https://<app-name>.fly.dev`). The server needs it to properly redirect users when logging in with OAuth providers like Google or GitHub.
* `JWT_SECRET` (required if using Wasp Auth)
You only need this environment variable if you're using Wasp's `auth` features. Set it to a random string at least 32 characters long (you can use an [online generator](https://djecrety.ir/)).
* `PORT`
The server's HTTP port number. This is where the server listens for requests (default: `3001`).
Using an external auth method?
If your app is using an external authentication method(s) supported by Wasp (such as [Google](https://wasp-lang.dev/docs/auth/social-auth/google#4-adding-environment-variables) or [GitHub](https://wasp-lang.dev/docs/auth/social-auth/github#4-adding-environment-variables)), make sure to additionally set the necessary environment variables specifically required by these method(s).
While these are the general instructions on deploying the server anywhere, we also have more detailed instructions for chosen providers below, so check that out for more guidance if you are deploying to one of those providers.
### 3\. Deploying the Web Client (frontend)[](#3-deploying-the-web-client-frontend "Direct link to 3. Deploying the Web Client (frontend)")
To build the web app, position yourself in `.wasp/build/web-app` directory:
Run
```
npm install && REACT_APP_API_URL=<url_to_wasp_backend> npm run build
```
where `<url_to_wasp_backend>` is the URL of the Wasp server that you previously deployed.
The command above will build the web client and put it in the `build/` directory in the `web-app` directory.
Since the app's frontend is just a bunch of static files, you can deploy it to any static hosting provider.
### 4\. Deploying the Database[](#4-deploying-the-database "Direct link to 4. Deploying the Database")
Any PostgreSQL database will do, as long as you provide the server with the correct `DATABASE_URL` env var and ensure that the database is accessible from the server.
## Different Providers[](#different-providers "Direct link to Different Providers")
We'll cover a few different deployment providers below:
* Fly.io (server and database)
* Netlify (client)
* Railway (server, client and database)
* Heroku (server and database)
## Fly.io (server and database)[](#flyio-server-and-database "Direct link to Fly.io (server and database)")
We will show how to deploy the server and provision a database for it on Fly.io.
We automated this process for you
If you want to do all of the work below with one command, you can use the [Wasp CLI](https://wasp-lang.dev/docs/advanced/deployment/cli#flyio).
Wasp CLI deploys the server, deploys the client, and sets up a database. It also gives you a way to redeploy (update) your app with a single command.
Fly.io offers a variety of free services that are perfect for deploying your first Wasp app! You will need a Fly.io account and the [`flyctl` CLI](https://fly.io/docs/hands-on/install-flyctl/).
note
Fly.io offers support for both locally built Docker containers and remotely built ones. However, for simplicity and reproducibility, we will default to the use of a remote Fly.io builder.
Additionally, `fly` is a symlink for `flyctl` on most systems and they can be used interchangeably.
Make sure you are logged in with `flyctl` CLI. You can check if you are logged in with `flyctl auth whoami`, and if you are not, you can log in with `flyctl auth login`.
### Set Up a Fly.io App[](#set-up-a-flyio-app "Direct link to Set Up a Fly.io App")
info
You need to do this only once per Wasp app.
Unless you already have a Fly.io app that you want to deploy to, let's create a new Fly.io app.
After you have [built the app](#1-generating-deployable-code), position yourself in `.wasp/build/` directory:
Next, run the launch command to set up a new app and create a `fly.toml` file:
```
flyctl launch --remote-only
```
This will ask you a series of questions, such as asking you to choose a region and whether you'd like a database.
* Say **yes** to **Would you like to set up a PostgreSQL database now?** and select **Development**. Fly.io will set a `DATABASE_URL` for you.
* Say **no** to **Would you like to deploy now?** (and to any additional questions).
We still need to set up several environment variables.
What if the database setup fails?
If your attempts to initiate a new app fail for whatever reason, then you should run `flyctl apps destroy <app-name>` before trying again. Fly does not allow you to create multiple apps with the same name.
What does it look like when your DB is deployed correctly?
Next, let's copy the `fly.toml` file up to our Wasp project dir for safekeeping.
Next, let's add a few more environment variables:
```
flyctl secrets set PORT=8080flyctl secrets set JWT_SECRET=<random_string_at_least_32_characters_long>flyctl secrets set WASP_WEB_CLIENT_URL=<url_of_where_client_will_be_deployed>flyctl secrets set WASP_SERVER_URL=<url_of_where_server_will_be_deployed>
```
note
If you do not know what your client URL is yet, don't worry. You can set `WASP_WEB_CLIENT_URL` after you deploy your client.
Using an external auth method?
If your app is using an external authentication method(s) supported by Wasp (such as [Google](https://wasp-lang.dev/docs/auth/social-auth/google#4-adding-environment-variables) or [GitHub](https://wasp-lang.dev/docs/auth/social-auth/github#4-adding-environment-variables)), make sure to additionally set the necessary environment variables specifically required by these method(s).
If you want to make sure you've added your secrets correctly, run `flyctl secrets list` in the terminal. Note that you will see hashed versions of your secrets to protect your sensitive data.
### Deploy to a Fly.io App[](#deploy-to-a-flyio-app "Direct link to Deploy to a Fly.io App")
While still in the `.wasp/build/` directory, run:
```
flyctl deploy --remote-only --config ../../fly.toml
```
This will build and deploy the backend of your Wasp app on Fly.io to `https://<app-name>.fly.dev` 🤘🎸
Now, if you haven't, you can deploy your client and add the client URL by running `flyctl secrets set WASP_WEB_CLIENT_URL=<url_of_deployed_client>`. We suggest using [Netlify](#netlify) for your client, but you can use any static hosting provider.
Additionally, some useful `flyctl` commands:
```
flyctl logsflyctl secrets listflyctl ssh console
```
### Redeploying After Wasp Builds[](#redeploying-after-wasp-builds "Direct link to Redeploying After Wasp Builds")
When you rebuild your Wasp app (with `wasp build`), it will remove your `.wasp/build/` directory. In there, you may have a `fly.toml` from any prior Fly.io deployments.
While we will improve this process in the future, in the meantime, you have a few options:
1. Copy the `fly.toml` file to a versioned directory, like your Wasp project dir.
From there, you can reference it in `flyctl deploy --config <path>` commands, like above.
2. Backup the `fly.toml` file somewhere before running `wasp build`, and copy it into .wasp/build/ after.
When the `fly.toml` file exists in .wasp/build/ dir, you do not need to specify the `--config <path>`.
3. Run `flyctl config save -a <app-name>` to regenerate the `fly.toml` file from the remote state stored in Fly.io.
## Netlify (client)[](#netlify-client "Direct link to Netlify (client)")
We'll show how to deploy the client on Netlify.
Netlify is a static hosting solution that is free for many use cases. You will need a Netlify account and [Netlify CLI](https://docs.netlify.com/cli/get-started/) installed to follow these instructions.
Make sure you are logged in with Netlify CLI. You can check if you are logged in with `netlify status`, and if you are not, you can log in with `netlify login`.
First, make sure you have [built the Wasp app](#1-generating-deployable-code). We'll build the client web app next.
To build the web app, position yourself in `.wasp/build/web-app` directory:
Run
```
npm install && REACT_APP_API_URL=<url_to_wasp_backend> npm run build
```
where `<url_to_wasp_backend>` is the URL of the Wasp server that you previously deployed.
We can now deploy the client with:
Carefully follow the instructions i.e. do you want to create a new app or use an existing one, the team under which your app will reside etc.
The final step is to run:
That is it! Your client should be live at `https://<app-name>.netlify.app` ✨
note
Make sure you set this URL as the `WASP_WEB_CLIENT_URL` environment variable in your server hosting environment (e.g., Fly.io or Heroku).
## Railway (server, client and database)[](#railway-server-client-and-database "Direct link to Railway (server, client and database)")
We will show how to deploy the client, the server, and provision a database on Railway.
Railway is a simple and great way to host your server and database. It's also possible to deploy your entire app: database, server, and client. You can use the platform for free for a limited time, or if you meet certain eligibility requirements. See their [plans page](https://docs.railway.app/reference/plans) for more info.
### Prerequisites[](#prerequisites "Direct link to Prerequisites")
To get started, follow these steps:
1. Make sure your Wasp app is built by running `wasp build` in the project dir.
2. Create a [Railway](https://railway.app/) account
Free Tier
Sign up with your GitHub account to be eligible for the free tier
3. Install the [Railway CLI](https://docs.railway.app/develop/cli#installation)
4. Run `railway login` and a browser tab will open to authenticate you.
### Create New Project[](#create-new-project "Direct link to Create New Project")
Let's create our Railway project:
1. Go to your [Railway dashboard](https://railway.app/dashboard), click on **New Project**, and select `Provision PostgreSQL` from the dropdown menu.
2. Once it initializes, right-click on the **New** button in the top right corner and select **Empty Service**.
3. Once it initializes, click on it, go to **Settings > General** and change the name to `server`
4. Go ahead and create another empty service and name it `client`
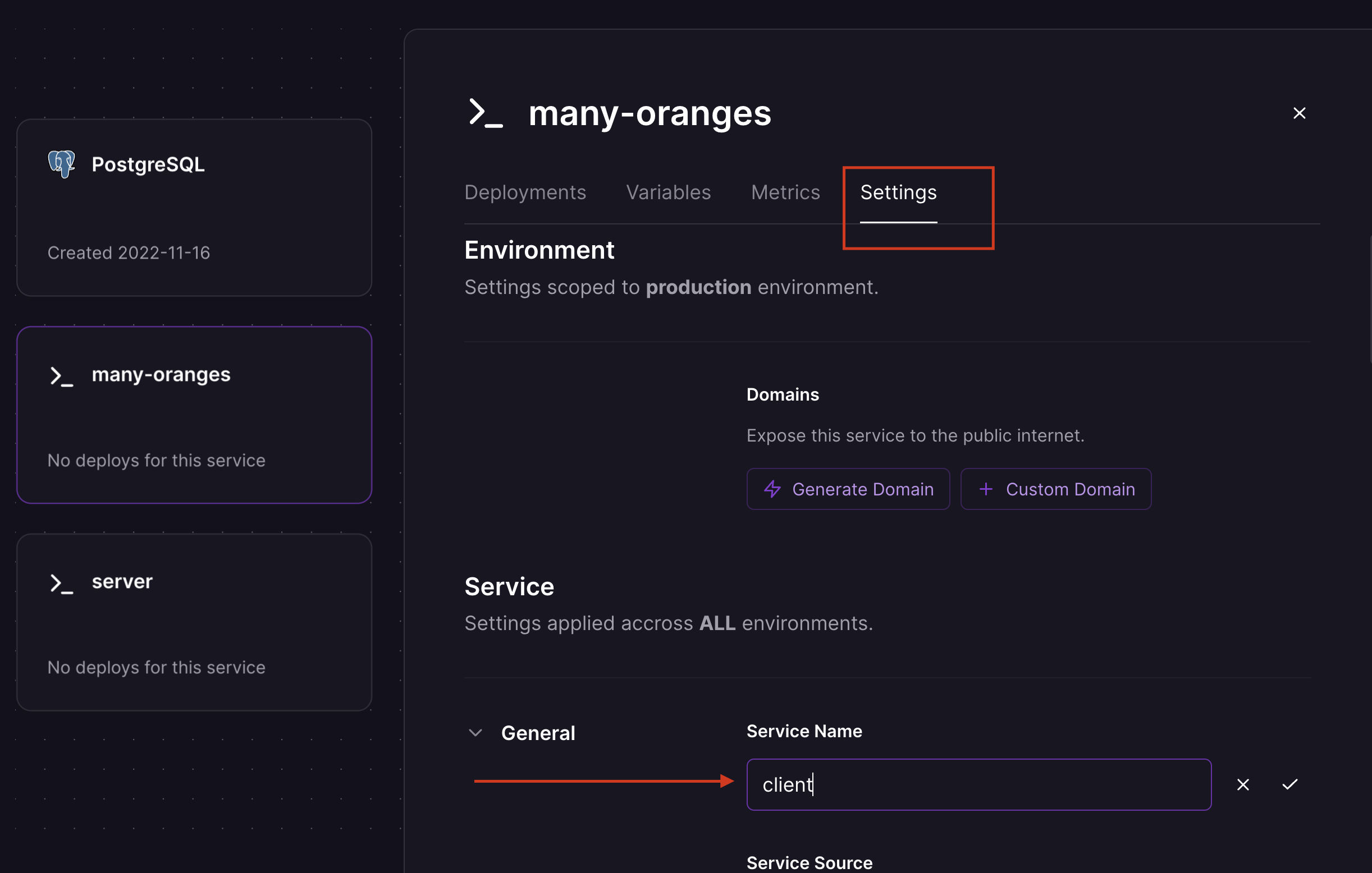
### Deploy Your App to Railway[](#deploy-your-app-to-railway "Direct link to Deploy Your App to Railway")
#### Setup Domains[](#setup-domains "Direct link to Setup Domains")
We'll need the domains for both the `server` and `client` services:
1. Go to the `server` instance's `Settings` tab, and click `Generate Domain`.
2. Do the same under the `client`'s `Settings`.
Copy the domains as we will need them later.
#### Deploying the Server[](#deploying-the-server "Direct link to Deploying the Server")
Let's deploy our server first:
1. Move into your app's `.wasp/build/` directory:
2. Link your app build to your newly created Railway project:
3. Go into the Railway dashboard and set up the required env variables:
Open the `Settings` and go to the `Variables` tab:
* click **Variable reference** and select `DATABASE_URL` (it will populate it with the correct value)
* add `WASP_WEB_CLIENT_URL` - enter the `client` domain (e.g. `https://client-production-XXXX.up.railway.app`)
* add `WASP_SERVER_URL` - enter the `server` domain (e.g. `https://server-production-XXXX.up.railway.app`)
* add `JWT_SECRET` - enter a random string at least 32 characters long (use an [online generator](https://djecrety.ir/))
Using an external auth method?
If your app is using an external authentication method(s) supported by Wasp (such as [Google](https://wasp-lang.dev/docs/auth/social-auth/google#4-adding-environment-variables) or [GitHub](https://wasp-lang.dev/docs/auth/social-auth/github#4-adding-environment-variables)), make sure to additionally set the necessary environment variables specifically required by these method(s).
4. Push and deploy the project:
Select `server` when prompted with `Select Service`.
Railway will now locate the Dockerfile and deploy your server 👍
#### Deploying the Client[](#deploying-the-client "Direct link to Deploying the Client")
1. Next, change into your app's frontend build directory `.wasp/build/web-app`:
2. Create the production build, using the `server` domain as the `REACT_APP_API_URL`:
```
npm install && REACT_APP_API_URL=<url_to_wasp_backend> npm run build
```
3. Next, we want to link this specific frontend directory to our project as well:
4. We need to configure Railway's static hosting for our client.
Setting Up Static Hosting
Copy the `build` folder within the `web-app` directory to `dist`:
We'll need to create the following files:
* `Dockerfile` with:
Dockerfile
```
FROM pierrezemb/gostaticCMD [ "-fallback", "index.html" ]COPY ./dist/ /srv/http/
```
* `.dockerignore` with:
You'll need to repeat these steps **each time** you run `wasp build` as it will remove the `.wasp/build/web-app` directory.
Here's a useful shell script to do the process
If you want to automate the process, save the following as `deploy_client.sh` in the root of your project:
deploy\_client.sh
```
#!/usr/bin/env bashif [ -z "$REACT_APP_API_URL" ]then echo "REACT_APP_API_URL is not set" exit 1fiwasp buildcd .wasp/build/web-appnpm install && REACT_APP_API_URL=$REACT_APP_API_URL npm run buildcp -r build distdockerfile_contents=$(cat <<EOFFROM pierrezemb/gostaticCMD [ "-fallback", "index.html" ]COPY ./dist/ /srv/http/EOF)dockerignore_contents=$(cat <<EOFnode_modules/EOF)echo "$dockerfile_contents" > Dockerfileecho "$dockerignore_contents" > .dockerignorerailway up
```
Make it executable with:
```
chmod +x deploy_client.sh
```
You can run it with:
```
REACT_APP_API_URL=<url_to_wasp_backend> ./deploy_client.sh
```
5. Set the `PORT` environment variable to `8043` under the `Variables` tab.
6. Deploy the client and select `client` when prompted with `Select Service`:
#### Conclusion[](#conclusion "Direct link to Conclusion")
And now your Wasp should be deployed! 🐝 🚂 🚀
Back in your [Railway dashboard](https://railway.app/dashboard), click on your project and you should see your newly deployed services: PostgreSQL, Server, and Client.
### Updates & Redeploying[](#updates--redeploying "Direct link to Updates & Redeploying")
When you make updates and need to redeploy:
* run `wasp build` to rebuild your app
* run `railway up` in the `.wasp/build` directory (server)
* repeat all the steps in the `.wasp/build/web-app` directory (client)
## Heroku (server and database)[](#heroku-server-and-database "Direct link to Heroku (server and database)")
We will show how to deploy the server and provision a database for it on Heroku.
note
Heroku used to offer free apps under certain limits. However, as of November 28, 2022, they ended support for their free tier. [https://blog.heroku.com/next-chapter](https://blog.heroku.com/next-chapter)
As such, we recommend using an alternative provider like [Fly.io](#flyio) for your first apps.
You will need Heroku account, `heroku` [CLI](https://devcenter.heroku.com/articles/heroku-cli) and `docker` CLI installed to follow these instructions.
Make sure you are logged in with `heroku` CLI. You can check if you are logged in with `heroku whoami`, and if you are not, you can log in with `heroku login`.
### Set Up a Heroku App[](#set-up-a-heroku-app "Direct link to Set Up a Heroku App")
info
You need to do this only once per Wasp app.
Unless you want to deploy to an existing Heroku app, let's create a new Heroku app:
Unless you have an external PostgreSQL database that you want to use, let's create a new database on Heroku and attach it to our app:
```
heroku addons:create --app <app-name> heroku-postgresql:mini
```
caution
Heroku does not offer a free plan anymore and `mini` is their cheapest database instance - it costs $5/mo.
Heroku will also set `DATABASE_URL` env var for us at this point. If you are using an external database, you will have to set it up yourself.
The `PORT` env var will also be provided by Heroku, so the ones left to set are the `JWT_SECRET`, `WASP_WEB_CLIENT_URL` and `WASP_SERVER_URL` env vars:
```
heroku config:set --app <app-name> JWT_SECRET=<random_string_at_least_32_characters_long>heroku config:set --app <app-name> WASP_WEB_CLIENT_URL=<url_of_where_client_will_be_deployed>heroku config:set --app <app-name> WASP_SERVER_URL=<url_of_where_server_will_be_deployed>
```
note
If you do not know what your client URL is yet, don't worry. You can set `WASP_WEB_CLIENT_URL` after you deploy your client.
### Deploy to a Heroku App[](#deploy-to-a-heroku-app "Direct link to Deploy to a Heroku App")
After you have [built the app](#1-generating-deployable-code), position yourself in `.wasp/build/` directory:
assuming you were at the root of your Wasp project at that moment.
Log in to Heroku Container Registry:
Build the docker image and push it to Heroku:
```
heroku container:push --app <app-name> web
```
App is still not deployed at this point. This step might take some time, especially the very first time, since there are no cached docker layers.
Note for Apple Silicon Users
Apple Silicon users need to build a non-Arm image, so the above step will not work at this time. Instead of `heroku container:push`, users instead should:
```
docker buildx build --platform linux/amd64 -t <app-name> .docker tag <app-name> registry.heroku.com/<app-name>/webdocker push registry.heroku.com/<app-name>/web
```
You are now ready to proceed to the next step.
Deploy the pushed image and restart the app:
```
heroku container:release --app <app-name> web
```
This is it, the backend is deployed at `https://<app-name>-XXXX.herokuapp.com` 🎉
Find out the exact app URL with:
```
heroku info --app <app-name>
```
Additionally, you can check out the logs with:
```
heroku logs --tail --app <app-name>
```
Using `pg-boss` with Heroku
If you wish to deploy an app leveraging [Jobs](https://wasp-lang.dev/docs/advanced/jobs) that use `pg-boss` as the executor to Heroku, you need to set an additional environment variable called `PG_BOSS_NEW_OPTIONS` to `{"connectionString":"<REGULAR_HEROKU_DATABASE_URL>","ssl":{"rejectUnauthorized":false}}`. This is because pg-boss uses the `pg` extension, which does not seem to connect to Heroku over SSL by default, which Heroku requires. Additionally, Heroku uses a self-signed cert, so we must handle that as well.
Read more: [https://devcenter.heroku.com/articles/connecting-heroku-postgres#connecting-in-node-js](https://devcenter.heroku.com/articles/connecting-heroku-postgres#connecting-in-node-js) | null | https://wasp-lang.dev/docs/advanced/deployment/manually | This document explains how to build and prepare your Wasp app for deployment. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece39cf96823f-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:50 GMT | Tue, 23 Apr 2024 15:24:50 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=KOHquSjkm1YY5Yzdy4JZ46KovJUKAScN7GA7KzDNHFgSh0Gzw6Eyw%2Fa%2Fb6zFfvEFjleODkHnV6jXdk%2FOQF5AkYpFVmIxeMp%2Fq8sbO77N9m%2FYp%2BGajvvihVS6slA5ZQ%2Fv"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 10de5279ea2bd2679153c2d067440bb2e157eb55 | null | 2588:1353DF:3C85685:47A23CC:6627D06A | HIT | MISS | cache-iad-kiad7000022-IAD | S1713885291.552604,VS0,VE34 | null | null | en | og:image | https://wasp-lang.dev/docs/advanced/deployment/manually | og:url | Deploying Manually | Wasp | og:title | This document explains how to build and prepare your Wasp app for deployment. | og:description | Deploying Manually | Wasp | null | Deploying Manually
This document explains how to build and prepare your Wasp app for deployment. You can then deploy the built Wasp app wherever and however you want, as long as your provider/server supports Wasp's build format.
After going through the general steps that apply to all deployments, you can follow step-by-step guides for deploying your Wasp app to the most popular providers:
Fly.io
Netlify
Railway
Heroku
No worries, you can still deploy your app if your desired provider isn't on the list - it just means we don't yet have a step-by-step guide for you to follow. Feel free to open a PR if you'd like to write one yourself :)
Deploying a Wasp App
Deploying a Wasp app comes down to the following:
Generating deployable code.
Deploying the API server (backend).
Deploying the web client (frontend).
Deploying a PostgreSQL database and keeping it running.
Let's go through each of these steps.
1. Generating Deployable Code
Running the command wasp build generates deployable code for the whole app in the .wasp/build/ directory.
PostgreSQL in production
You won't be able to build the app if you are using SQLite as a database (which is the default database). You'll have to switch to PostgreSQL before deploying to production.
2. Deploying the API Server (backend)
There's a Dockerfile that defines an image for building the server in the .wasp/build directory.
To run the server in production, deploy this Docker image to a hosting provider and ensure the required environment variables on the provider are correctly set up (the mechanism of setting these up is specific per provider). All necessary environment variables are listed in the next section.
Environment Variables
Here are the environment variables your server will be looking for:
DATABASE_URL required
The URL of the PostgreSQL database you want your app to use (e.g., postgresql://mydbuser:mypass@localhost:5432/nameofmydb).
WASP_WEB_CLIENT_URL required
The URL where you plan to deploy your frontend app is running (e.g., https://<app-name>.netlify.app). The server needs to know about it to properly configure Same-Origin Policy (CORS) headers.
WASP_SERVER_URL required
The URL where the server is running (e.g., https://<app-name>.fly.dev). The server needs it to properly redirect users when logging in with OAuth providers like Google or GitHub.
JWT_SECRET (required if using Wasp Auth)
You only need this environment variable if you're using Wasp's auth features. Set it to a random string at least 32 characters long (you can use an online generator).
PORT
The server's HTTP port number. This is where the server listens for requests (default: 3001).
Using an external auth method?
If your app is using an external authentication method(s) supported by Wasp (such as Google or GitHub), make sure to additionally set the necessary environment variables specifically required by these method(s).
While these are the general instructions on deploying the server anywhere, we also have more detailed instructions for chosen providers below, so check that out for more guidance if you are deploying to one of those providers.
3. Deploying the Web Client (frontend)
To build the web app, position yourself in .wasp/build/web-app directory:
Run
npm install && REACT_APP_API_URL=<url_to_wasp_backend> npm run build
where <url_to_wasp_backend> is the URL of the Wasp server that you previously deployed.
The command above will build the web client and put it in the build/ directory in the web-app directory.
Since the app's frontend is just a bunch of static files, you can deploy it to any static hosting provider.
4. Deploying the Database
Any PostgreSQL database will do, as long as you provide the server with the correct DATABASE_URL env var and ensure that the database is accessible from the server.
Different Providers
We'll cover a few different deployment providers below:
Fly.io (server and database)
Netlify (client)
Railway (server, client and database)
Heroku (server and database)
Fly.io (server and database)
We will show how to deploy the server and provision a database for it on Fly.io.
We automated this process for you
If you want to do all of the work below with one command, you can use the Wasp CLI.
Wasp CLI deploys the server, deploys the client, and sets up a database. It also gives you a way to redeploy (update) your app with a single command.
Fly.io offers a variety of free services that are perfect for deploying your first Wasp app! You will need a Fly.io account and the flyctl CLI.
note
Fly.io offers support for both locally built Docker containers and remotely built ones. However, for simplicity and reproducibility, we will default to the use of a remote Fly.io builder.
Additionally, fly is a symlink for flyctl on most systems and they can be used interchangeably.
Make sure you are logged in with flyctl CLI. You can check if you are logged in with flyctl auth whoami, and if you are not, you can log in with flyctl auth login.
Set Up a Fly.io App
info
You need to do this only once per Wasp app.
Unless you already have a Fly.io app that you want to deploy to, let's create a new Fly.io app.
After you have built the app, position yourself in .wasp/build/ directory:
Next, run the launch command to set up a new app and create a fly.toml file:
flyctl launch --remote-only
This will ask you a series of questions, such as asking you to choose a region and whether you'd like a database.
Say yes to Would you like to set up a PostgreSQL database now? and select Development. Fly.io will set a DATABASE_URL for you.
Say no to Would you like to deploy now? (and to any additional questions).
We still need to set up several environment variables.
What if the database setup fails?
If your attempts to initiate a new app fail for whatever reason, then you should run flyctl apps destroy <app-name> before trying again. Fly does not allow you to create multiple apps with the same name.
What does it look like when your DB is deployed correctly?
Next, let's copy the fly.toml file up to our Wasp project dir for safekeeping.
Next, let's add a few more environment variables:
flyctl secrets set PORT=8080
flyctl secrets set JWT_SECRET=<random_string_at_least_32_characters_long>
flyctl secrets set WASP_WEB_CLIENT_URL=<url_of_where_client_will_be_deployed>
flyctl secrets set WASP_SERVER_URL=<url_of_where_server_will_be_deployed>
note
If you do not know what your client URL is yet, don't worry. You can set WASP_WEB_CLIENT_URL after you deploy your client.
Using an external auth method?
If your app is using an external authentication method(s) supported by Wasp (such as Google or GitHub), make sure to additionally set the necessary environment variables specifically required by these method(s).
If you want to make sure you've added your secrets correctly, run flyctl secrets list in the terminal. Note that you will see hashed versions of your secrets to protect your sensitive data.
Deploy to a Fly.io App
While still in the .wasp/build/ directory, run:
flyctl deploy --remote-only --config ../../fly.toml
This will build and deploy the backend of your Wasp app on Fly.io to https://<app-name>.fly.dev 🤘🎸
Now, if you haven't, you can deploy your client and add the client URL by running flyctl secrets set WASP_WEB_CLIENT_URL=<url_of_deployed_client>. We suggest using Netlify for your client, but you can use any static hosting provider.
Additionally, some useful flyctl commands:
flyctl logs
flyctl secrets list
flyctl ssh console
Redeploying After Wasp Builds
When you rebuild your Wasp app (with wasp build), it will remove your .wasp/build/ directory. In there, you may have a fly.toml from any prior Fly.io deployments.
While we will improve this process in the future, in the meantime, you have a few options:
Copy the fly.toml file to a versioned directory, like your Wasp project dir.
From there, you can reference it in flyctl deploy --config <path> commands, like above.
Backup the fly.toml file somewhere before running wasp build, and copy it into .wasp/build/ after.
When the fly.toml file exists in .wasp/build/ dir, you do not need to specify the --config <path>.
Run flyctl config save -a <app-name> to regenerate the fly.toml file from the remote state stored in Fly.io.
Netlify (client)
We'll show how to deploy the client on Netlify.
Netlify is a static hosting solution that is free for many use cases. You will need a Netlify account and Netlify CLI installed to follow these instructions.
Make sure you are logged in with Netlify CLI. You can check if you are logged in with netlify status, and if you are not, you can log in with netlify login.
First, make sure you have built the Wasp app. We'll build the client web app next.
To build the web app, position yourself in .wasp/build/web-app directory:
Run
npm install && REACT_APP_API_URL=<url_to_wasp_backend> npm run build
where <url_to_wasp_backend> is the URL of the Wasp server that you previously deployed.
We can now deploy the client with:
Carefully follow the instructions i.e. do you want to create a new app or use an existing one, the team under which your app will reside etc.
The final step is to run:
That is it! Your client should be live at https://<app-name>.netlify.app ✨
note
Make sure you set this URL as the WASP_WEB_CLIENT_URL environment variable in your server hosting environment (e.g., Fly.io or Heroku).
Railway (server, client and database)
We will show how to deploy the client, the server, and provision a database on Railway.
Railway is a simple and great way to host your server and database. It's also possible to deploy your entire app: database, server, and client. You can use the platform for free for a limited time, or if you meet certain eligibility requirements. See their plans page for more info.
Prerequisites
To get started, follow these steps:
Make sure your Wasp app is built by running wasp build in the project dir.
Create a Railway account
Free Tier
Sign up with your GitHub account to be eligible for the free tier
Install the Railway CLI
Run railway login and a browser tab will open to authenticate you.
Create New Project
Let's create our Railway project:
Go to your Railway dashboard, click on New Project, and select Provision PostgreSQL from the dropdown menu.
Once it initializes, right-click on the New button in the top right corner and select Empty Service.
Once it initializes, click on it, go to Settings > General and change the name to server
Go ahead and create another empty service and name it client
Deploy Your App to Railway
Setup Domains
We'll need the domains for both the server and client services:
Go to the server instance's Settings tab, and click Generate Domain.
Do the same under the client's Settings.
Copy the domains as we will need them later.
Deploying the Server
Let's deploy our server first:
Move into your app's .wasp/build/ directory:
Link your app build to your newly created Railway project:
Go into the Railway dashboard and set up the required env variables:
Open the Settings and go to the Variables tab:
click Variable reference and select DATABASE_URL (it will populate it with the correct value)
add WASP_WEB_CLIENT_URL - enter the client domain (e.g. https://client-production-XXXX.up.railway.app)
add WASP_SERVER_URL - enter the server domain (e.g. https://server-production-XXXX.up.railway.app)
add JWT_SECRET - enter a random string at least 32 characters long (use an online generator)
Using an external auth method?
If your app is using an external authentication method(s) supported by Wasp (such as Google or GitHub), make sure to additionally set the necessary environment variables specifically required by these method(s).
Push and deploy the project:
Select server when prompted with Select Service.
Railway will now locate the Dockerfile and deploy your server 👍
Deploying the Client
Next, change into your app's frontend build directory .wasp/build/web-app:
Create the production build, using the server domain as the REACT_APP_API_URL:
npm install && REACT_APP_API_URL=<url_to_wasp_backend> npm run build
Next, we want to link this specific frontend directory to our project as well:
We need to configure Railway's static hosting for our client.
Setting Up Static Hosting
Copy the build folder within the web-app directory to dist:
We'll need to create the following files:
Dockerfile with:
Dockerfile
FROM pierrezemb/gostatic
CMD [ "-fallback", "index.html" ]
COPY ./dist/ /srv/http/
.dockerignore with:
You'll need to repeat these steps each time you run wasp build as it will remove the .wasp/build/web-app directory.
Here's a useful shell script to do the process
If you want to automate the process, save the following as deploy_client.sh in the root of your project:
deploy_client.sh
#!/usr/bin/env bash
if [ -z "$REACT_APP_API_URL" ]
then
echo "REACT_APP_API_URL is not set"
exit 1
fi
wasp build
cd .wasp/build/web-app
npm install && REACT_APP_API_URL=$REACT_APP_API_URL npm run build
cp -r build dist
dockerfile_contents=$(cat <<EOF
FROM pierrezemb/gostatic
CMD [ "-fallback", "index.html" ]
COPY ./dist/ /srv/http/
EOF
)
dockerignore_contents=$(cat <<EOF
node_modules/
EOF
)
echo "$dockerfile_contents" > Dockerfile
echo "$dockerignore_contents" > .dockerignore
railway up
Make it executable with:
chmod +x deploy_client.sh
You can run it with:
REACT_APP_API_URL=<url_to_wasp_backend> ./deploy_client.sh
Set the PORT environment variable to 8043 under the Variables tab.
Deploy the client and select client when prompted with Select Service:
Conclusion
And now your Wasp should be deployed! 🐝 🚂 🚀
Back in your Railway dashboard, click on your project and you should see your newly deployed services: PostgreSQL, Server, and Client.
Updates & Redeploying
When you make updates and need to redeploy:
run wasp build to rebuild your app
run railway up in the .wasp/build directory (server)
repeat all the steps in the .wasp/build/web-app directory (client)
Heroku (server and database)
We will show how to deploy the server and provision a database for it on Heroku.
note
Heroku used to offer free apps under certain limits. However, as of November 28, 2022, they ended support for their free tier. https://blog.heroku.com/next-chapter
As such, we recommend using an alternative provider like Fly.io for your first apps.
You will need Heroku account, heroku CLI and docker CLI installed to follow these instructions.
Make sure you are logged in with heroku CLI. You can check if you are logged in with heroku whoami, and if you are not, you can log in with heroku login.
Set Up a Heroku App
info
You need to do this only once per Wasp app.
Unless you want to deploy to an existing Heroku app, let's create a new Heroku app:
Unless you have an external PostgreSQL database that you want to use, let's create a new database on Heroku and attach it to our app:
heroku addons:create --app <app-name> heroku-postgresql:mini
caution
Heroku does not offer a free plan anymore and mini is their cheapest database instance - it costs $5/mo.
Heroku will also set DATABASE_URL env var for us at this point. If you are using an external database, you will have to set it up yourself.
The PORT env var will also be provided by Heroku, so the ones left to set are the JWT_SECRET, WASP_WEB_CLIENT_URL and WASP_SERVER_URL env vars:
heroku config:set --app <app-name> JWT_SECRET=<random_string_at_least_32_characters_long>
heroku config:set --app <app-name> WASP_WEB_CLIENT_URL=<url_of_where_client_will_be_deployed>
heroku config:set --app <app-name> WASP_SERVER_URL=<url_of_where_server_will_be_deployed>
note
If you do not know what your client URL is yet, don't worry. You can set WASP_WEB_CLIENT_URL after you deploy your client.
Deploy to a Heroku App
After you have built the app, position yourself in .wasp/build/ directory:
assuming you were at the root of your Wasp project at that moment.
Log in to Heroku Container Registry:
Build the docker image and push it to Heroku:
heroku container:push --app <app-name> web
App is still not deployed at this point. This step might take some time, especially the very first time, since there are no cached docker layers.
Note for Apple Silicon Users
Apple Silicon users need to build a non-Arm image, so the above step will not work at this time. Instead of heroku container:push, users instead should:
docker buildx build --platform linux/amd64 -t <app-name> .
docker tag <app-name> registry.heroku.com/<app-name>/web
docker push registry.heroku.com/<app-name>/web
You are now ready to proceed to the next step.
Deploy the pushed image and restart the app:
heroku container:release --app <app-name> web
This is it, the backend is deployed at https://<app-name>-XXXX.herokuapp.com 🎉
Find out the exact app URL with:
heroku info --app <app-name>
Additionally, you can check out the logs with:
heroku logs --tail --app <app-name>
Using pg-boss with Heroku
If you wish to deploy an app leveraging Jobs that use pg-boss as the executor to Heroku, you need to set an additional environment variable called PG_BOSS_NEW_OPTIONS to {"connectionString":"<REGULAR_HEROKU_DATABASE_URL>","ssl":{"rejectUnauthorized":false}}. This is because pg-boss uses the pg extension, which does not seem to connect to Heroku over SSL by default, which Heroku requires. Additionally, Heroku uses a self-signed cert, so we must handle that as well.
Read more: https://devcenter.heroku.com/articles/connecting-heroku-postgres#connecting-in-node-js | https://wasp-lang.dev/docs/advanced/deployment/manually |
|
1 | null | 2024-04-23T15:14:53.326Z | https://wasp-lang.dev/docs/advanced/email | https://wasp-lang.dev/docs | http | ## Sending Emails
With Wasp's email-sending feature, you can easily integrate email functionality into your web application.
* JavaScript
* TypeScript
main.wasp
```
app Example { ... emailSender: { provider: <provider>, defaultFrom: { name: "Example", email: "[email protected]" }, }}
```
Choose from one of the providers:
* `Dummy` (development only),
* `Mailgun`,
* `SendGrid`
* or the good old `SMTP`.
Optionally, define the `defaultFrom` field, so you don't need to provide it whenever sending an email.
## Sending Emails[](#sending-emails-1 "Direct link to Sending Emails")
Before jumping into details about setting up various providers, let's see how easy it is to send emails.
You import the `emailSender` that is provided by the `wasp/server/email` module and call the `send` method on it.
* JavaScript
* TypeScript
src/actions/sendEmail.js
```
import { emailSender } from "wasp/server/email";// In some action handler...const info = await emailSender.send({ from: { name: "John Doe", email: "[email protected]", }, to: "[email protected]", subject: "Saying hello", text: "Hello world", html: "Hello <strong>world</strong>",});
```
Read more about the `send` method in the [API Reference](#javascript-api).
The `send` method returns an object with the status of the sent email. It varies depending on the provider you use.
## Providers[](#providers "Direct link to Providers")
We'll go over all of the available providers in the next section. For some of them, you'll need to set up some env variables. You can do that in the `.env.server` file.
### Using the Dummy Provider[](#using-the-dummy-provider "Direct link to Using the Dummy Provider")
Dummy Provider is not for production use
The `Dummy` provider is not for production use. It is only meant to be used during development. If you try building your app with the `Dummy` provider, the build will fail.
To speed up development, Wasp offers a `Dummy` email sender that `console.log`s the emails in the console. Since it doesn't send emails for real, it doesn't require any setup.
Set the provider to `Dummy` in your `main.wasp` file.
* JavaScript
* TypeScript
main.wasp
```
app Example { ... emailSender: { provider: Dummy, }}
```
### Using the SMTP Provider[](#using-the-smtp-provider "Direct link to Using the SMTP Provider")
First, set the provider to `SMTP` in your `main.wasp` file.
* JavaScript
* TypeScript
main.wasp
```
app Example { ... emailSender: { provider: SMTP, }}
```
Then, add the following env variables to your `.env.server` file.
.env.server
```
SMTP_HOST=SMTP_USERNAME=SMTP_PASSWORD=SMTP_PORT=
```
Many transactional email providers (e.g. Mailgun, SendGrid but also others) can also use SMTP, so you can use them as well.
### Using the Mailgun Provider[](#using-the-mailgun-provider "Direct link to Using the Mailgun Provider")
Set the provider to `Mailgun` in the `main.wasp` file.
* JavaScript
* TypeScript
main.wasp
```
app Example { ... emailSender: { provider: Mailgun, }}
```
Then, get the Mailgun API key and domain and add them to your `.env.server` file.
#### Getting the API Key and Domain[](#getting-the-api-key-and-domain "Direct link to Getting the API Key and Domain")
1. Go to [Mailgun](https://www.mailgun.com/) and create an account.
2. Go to [API Keys](https://app.mailgun.com/app/account/security/api_keys) and create a new API key.
3. Copy the API key and add it to your `.env.server` file.
4. Go to [Domains](https://app.mailgun.com/app/domains) and create a new domain.
5. Copy the domain and add it to your `.env.server` file.
.env.server
```
MAILGUN_API_KEY=MAILGUN_DOMAIN=
```
### Using the SendGrid Provider[](#using-the-sendgrid-provider "Direct link to Using the SendGrid Provider")
Set the provider field to `SendGrid` in your `main.wasp` file.
* JavaScript
* TypeScript
main.wasp
```
app Example { ... emailSender: { provider: SendGrid, }}
```
Then, get the SendGrid API key and add it to your `.env.server` file.
#### Getting the API Key[](#getting-the-api-key "Direct link to Getting the API Key")
1. Go to [SendGrid](https://sendgrid.com/) and create an account.
2. Go to [API Keys](https://app.sendgrid.com/settings/api_keys) and create a new API key.
3. Copy the API key and add it to your `.env.server` file.
## API Reference[](#api-reference "Direct link to API Reference")
### `emailSender` dict[](#emailsender-dict "Direct link to emailsender-dict")
* JavaScript
* TypeScript
main.wasp
```
app Example { ... emailSender: { provider: <provider>, defaultFrom: { name: "Example", email: "[email protected]" }, }}
```
The `emailSender` dict has the following fields:
* `provider: Provider` required
The provider you want to use. Choose from `Dummy`, `SMTP`, `Mailgun` or `SendGrid`.
Dummy Provider is not for production use
The `Dummy` provider is not for production use. It is only meant to be used during development. If you try building your app with the `Dummy` provider, the build will fail.
* `defaultFrom: dict`
The default sender's details. If you set this field, you don't need to provide the `from` field when sending an email.
### JavaScript API[](#javascript-api "Direct link to JavaScript API")
Using the `emailSender` in :
* JavaScript
* TypeScript
src/actions/sendEmail.js
```
import { emailSender } from "wasp/server/email";// In some action handler...const info = await emailSender.send({ from: { name: "John Doe", email: "[email protected]", }, to: "[email protected]", subject: "Saying hello", text: "Hello world", html: "Hello <strong>world</strong>",});
```
The `send` method accepts an object with the following fields:
* `from: object`
The sender's details. If you set up `defaultFrom` field in the `emailSender` dict in Wasp file, this field is optional.
* `name: string`
The name of the sender.
* `email: string`
The email address of the sender.
* `to: string` required
The recipient's email address.
* `subject: string` required
The subject of the email.
* `text: string` required
The text version of the email.
* `html: string` required
The HTML version of the email | null | https://wasp-lang.dev/docs/advanced/email | With Wasp's email-sending feature, you can easily integrate email functionality into your web application. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece43ac1c3b12-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:52 GMT | Tue, 23 Apr 2024 15:24:52 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=fcOg4%2FQALkPq0mLecpv1LWct2m7p%2BDTWWu7rZyyIAm18%2FExbzJ4%2BlRM0O8AsOxT16myhhQowDrTcN9hO3pU%2BQn99KAbg7iw1CSIz%2FPO9A2OiEhxpn5G48PCelh9tRSrf"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 317a1c8fe799b96c7b96496984e37fac6e45b285 | null | ABA2:1726:4F6475:5F73D7:6627D06B | HIT | MISS | cache-iad-kiad7000045-IAD | S1713885292.133789,VS0,VE13 | null | null | en | og:image | https://wasp-lang.dev/docs/advanced/email | og:url | Sending Emails | Wasp | og:title | With Wasp's email-sending feature, you can easily integrate email functionality into your web application. | og:description | Sending Emails | Wasp | null | Sending Emails
With Wasp's email-sending feature, you can easily integrate email functionality into your web application.
JavaScript
TypeScript
main.wasp
app Example {
...
emailSender: {
provider: <provider>,
defaultFrom: {
name: "Example",
email: "[email protected]"
},
}
}
Choose from one of the providers:
Dummy (development only),
Mailgun,
SendGrid
or the good old SMTP.
Optionally, define the defaultFrom field, so you don't need to provide it whenever sending an email.
Sending Emails
Before jumping into details about setting up various providers, let's see how easy it is to send emails.
You import the emailSender that is provided by the wasp/server/email module and call the send method on it.
JavaScript
TypeScript
src/actions/sendEmail.js
import { emailSender } from "wasp/server/email";
// In some action handler...
const info = await emailSender.send({
from: {
name: "John Doe",
email: "[email protected]",
},
to: "[email protected]",
subject: "Saying hello",
text: "Hello world",
html: "Hello <strong>world</strong>",
});
Read more about the send method in the API Reference.
The send method returns an object with the status of the sent email. It varies depending on the provider you use.
Providers
We'll go over all of the available providers in the next section. For some of them, you'll need to set up some env variables. You can do that in the .env.server file.
Using the Dummy Provider
Dummy Provider is not for production use
The Dummy provider is not for production use. It is only meant to be used during development. If you try building your app with the Dummy provider, the build will fail.
To speed up development, Wasp offers a Dummy email sender that console.logs the emails in the console. Since it doesn't send emails for real, it doesn't require any setup.
Set the provider to Dummy in your main.wasp file.
JavaScript
TypeScript
main.wasp
app Example {
...
emailSender: {
provider: Dummy,
}
}
Using the SMTP Provider
First, set the provider to SMTP in your main.wasp file.
JavaScript
TypeScript
main.wasp
app Example {
...
emailSender: {
provider: SMTP,
}
}
Then, add the following env variables to your .env.server file.
.env.server
SMTP_HOST=
SMTP_USERNAME=
SMTP_PASSWORD=
SMTP_PORT=
Many transactional email providers (e.g. Mailgun, SendGrid but also others) can also use SMTP, so you can use them as well.
Using the Mailgun Provider
Set the provider to Mailgun in the main.wasp file.
JavaScript
TypeScript
main.wasp
app Example {
...
emailSender: {
provider: Mailgun,
}
}
Then, get the Mailgun API key and domain and add them to your .env.server file.
Getting the API Key and Domain
Go to Mailgun and create an account.
Go to API Keys and create a new API key.
Copy the API key and add it to your .env.server file.
Go to Domains and create a new domain.
Copy the domain and add it to your .env.server file.
.env.server
MAILGUN_API_KEY=
MAILGUN_DOMAIN=
Using the SendGrid Provider
Set the provider field to SendGrid in your main.wasp file.
JavaScript
TypeScript
main.wasp
app Example {
...
emailSender: {
provider: SendGrid,
}
}
Then, get the SendGrid API key and add it to your .env.server file.
Getting the API Key
Go to SendGrid and create an account.
Go to API Keys and create a new API key.
Copy the API key and add it to your .env.server file.
API Reference
emailSender dict
JavaScript
TypeScript
main.wasp
app Example {
...
emailSender: {
provider: <provider>,
defaultFrom: {
name: "Example",
email: "[email protected]"
},
}
}
The emailSender dict has the following fields:
provider: Provider required
The provider you want to use. Choose from Dummy, SMTP, Mailgun or SendGrid.
Dummy Provider is not for production use
The Dummy provider is not for production use. It is only meant to be used during development. If you try building your app with the Dummy provider, the build will fail.
defaultFrom: dict
The default sender's details. If you set this field, you don't need to provide the from field when sending an email.
JavaScript API
Using the emailSender in :
JavaScript
TypeScript
src/actions/sendEmail.js
import { emailSender } from "wasp/server/email";
// In some action handler...
const info = await emailSender.send({
from: {
name: "John Doe",
email: "[email protected]",
},
to: "[email protected]",
subject: "Saying hello",
text: "Hello world",
html: "Hello <strong>world</strong>",
});
The send method accepts an object with the following fields:
from: object
The sender's details. If you set up defaultFrom field in the emailSender dict in Wasp file, this field is optional.
name: string
The name of the sender.
email: string
The email address of the sender.
to: string required
The recipient's email address.
subject: string required
The subject of the email.
text: string required
The text version of the email.
html: string required
The HTML version of the email | https://wasp-lang.dev/docs/advanced/email |
|
1 | null | 2024-04-23T15:14:53.631Z | https://wasp-lang.dev/docs/advanced/accessing-app-config | https://wasp-lang.dev/docs | http | Whenever you start a Wasp app, you are starting two processes.
Check [the introduction](https://wasp-lang.dev/docs) for a more in-depth explanation of Wasp's runtime architecture.
You can configure both processes through environment variables. See [the deployment instructions](https://wasp-lang.dev/docs/advanced/deployment/manually#environment-variables) for a full list of supported variables.
Wasp gives you runtime access to the processes' configurations through **configuration objects**.
```
import { config } from 'wasp/server'console.log(config.frontendUrl)
``` | null | https://wasp-lang.dev/docs/advanced/accessing-app-config | Whenever you start a Wasp app, you are starting two processes. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece4cad0607cc-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:53 GMT | Tue, 23 Apr 2024 15:24:53 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=QUDsZ62mLvUZ7T%2F4sTGqAQE1Z3tvYiuFrRaP%2F%2FEogy%2FqLZeg14TSOU3n2%2B7RJdYeDZZ5v4QWDJLd2U62B5RPlQPQMrAkoYQBdHJUpsn4c6xef6rdEWVPRP2y3UbfbR6v"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 67c1f95e0f08a2db43b07f33d9777b9a269edfa5 | null | 44B0:209F3A:3A7FBD4:458637C:6627D06D | null | MISS | cache-iad-kiad7000128-IAD | S1713885294.597251,VS0,VE17 | null | null | en | og:image | https://wasp-lang.dev/docs/advanced/accessing-app-config | og:url | Accessing the configuration | Wasp | og:title | Whenever you start a Wasp app, you are starting two processes. | og:description | Accessing the configuration | Wasp | null | Whenever you start a Wasp app, you are starting two processes.
Check the introduction for a more in-depth explanation of Wasp's runtime architecture.
You can configure both processes through environment variables. See the deployment instructions for a full list of supported variables.
Wasp gives you runtime access to the processes' configurations through configuration objects.
import { config } from 'wasp/server'
console.log(config.frontendUrl) | https://wasp-lang.dev/docs/advanced/accessing-app-config |
|
1 | null | 2024-04-23T15:14:53.558Z | https://wasp-lang.dev/docs/advanced/jobs | https://wasp-lang.dev/docs | http | ## Recurring Jobs
In most web apps, users send requests to the server and receive responses with some data. When the server responds quickly, the app feels responsive and smooth.
What if the server needs extra time to fully process the request? This might mean sending an email or making a slow HTTP request to an external API. In that case, it's a good idea to respond to the user as soon as possible and do the remaining work in the background.
Wasp supports background jobs that can help you with this:
* Jobs persist between server restarts,
* Jobs can be retried if they fail,
* Jobs can be delayed until a future time,
* Jobs can have a recurring schedule.
## Using Jobs[](#using-jobs "Direct link to Using Jobs")
### Job Definition and Usage[](#job-definition-and-usage "Direct link to Job Definition and Usage")
Let's write an example Job that will print a message to the console and return a list of tasks from the database.
1. Start by creating a Job declaration in your `.wasp` file:
* JavaScript
* TypeScript
main.wasp
```
job mySpecialJob { executor: PgBoss, perform: { fn: import { foo } from "@src/workers/bar" }, entities: [Task],}
```
2. After declaring the Job, implement its worker function:
* JavaScript
* TypeScript
src/workers/bar.js
```
export const foo = async ({ name }, context) => { console.log(`Hello ${name}!`) const tasks = await context.entities.Task.findMany({}) return { tasks }}
```
The worker function
The worker function must be an `async` function. The function's return value represents the Job's result.
The worker function accepts two arguments:
* `args`: The data passed into the job when it's submitted.
* `context: { entities }`: The context object containing entities you put in the Job declaration.
3. After successfully defining the job, you can submit work to be done in your [Operations](https://wasp-lang.dev/docs/data-model/operations/overview) or [setupFn](https://wasp-lang.dev/docs/project/server-config#setup-function) (or any other NodeJS code):
* JavaScript
* TypeScript
someAction.js
```
import { mySpecialJob } from 'wasp/server/jobs'const submittedJob = await mySpecialJob.submit({ job: "Johnny" })// Or, if you'd prefer it to execute in the future, just add a .delay().// It takes a number of seconds, Date, or ISO date string.await mySpecialJob .delay(10) .submit({ name: "Johnny" })
```
And that's it. Your job will be executed by `PgBoss` as if you called `foo({ name: "Johnny" })`.
In our example, `foo` takes an argument, but passing arguments to jobs is not a requirement. It depends on how you've implemented your worker function.
### Recurring Jobs[](#recurring-jobs "Direct link to Recurring Jobs")
If you have work that needs to be done on some recurring basis, you can add a `schedule` to your job declaration:
* JavaScript
* TypeScript
main.wasp
```
job mySpecialJob { executor: PgBoss, perform: { fn: import { foo } from "@src/workers/bar" }, schedule: { cron: "0 * * * *", args: {=json { "job": "args" } json=} // optional }}
```
In this example, you _don't_ need to invoke anything in . You can imagine `foo({ job: "args" })` getting automatically scheduled and invoked for you every hour.
## API Reference[](#api-reference "Direct link to API Reference")
### Declaring Jobs[](#declaring-jobs "Direct link to Declaring Jobs")
* JavaScript
* TypeScript
main.wasp
```
job mySpecialJob { executor: PgBoss, perform: { fn: import { foo } from "@src/workers/bar", executorOptions: { pgBoss: {=json { "retryLimit": 1 } json=} } }, schedule: { cron: "*/5 * * * *", args: {=json { "foo": "bar" } json=}, executorOptions: { pgBoss: {=json { "retryLimit": 0 } json=} } }, entities: [Task],}
```
The Job declaration has the following fields:
* `executor: JobExecutor` required
Job executors
Our jobs need job executors to handle the _scheduling, monitoring, and execution_.
`PgBoss` is currently our only job executor, and is recommended for low-volume production use cases. It requires your `app.db.system` to be `PostgreSQL`.
We have selected [pg-boss](https://github.com/timgit/pg-boss/) as our first job executor to handle the low-volume, basic job queue workloads many web applications have. By using PostgreSQL (and [SKIP LOCKED](https://www.2ndquadrant.com/en/blog/what-is-select-skip-locked-for-in-postgresql-9-5/)) as its storage and synchronization mechanism, it allows us to provide many job queue pros without any additional infrastructure or complex management.
info
Keep in mind that pg-boss jobs run alongside your other server-side code, so they are not appropriate for CPU-heavy workloads. Additionally, some care is required if you modify scheduled jobs. Please see pg-boss details below for more information.
pg-boss details
pg-boss provides many useful features, which can be found [here](https://github.com/timgit/pg-boss/blob/8.4.2/README.md).
When you add pg-boss to a Wasp project, it will automatically add a new schema to your database called `pgboss` with some internal tracking tables, including `job` and `schedule`. pg-boss tables have a `name` column in most tables that will correspond to your Job identifier. Additionally, these tables maintain arguments, states, return values, retry information, start and expiration times, and other metadata required by pg-boss.
If you need to customize the creation of the pg-boss instance, you can set an environment variable called `PG_BOSS_NEW_OPTIONS` to a stringified JSON object containing [these initialization parameters](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#newoptions). **NOTE**: Setting this overwrites all Wasp defaults, so you must include database connection information as well.
### pg-boss considerations[](#pg-boss-considerations "Direct link to pg-boss considerations")
* Wasp starts pg-boss alongside your web server's application, where both are simultaneously operational. This means that jobs running via pg-boss and the rest of the server logic (like Operations) share the CPU, therefore you should avoid running CPU-intensive tasks via jobs.
* Wasp does not (yet) support independent, horizontal scaling of pg-boss-only applications, nor starting them as separate workers/processes/threads.
* The job name/identifier in your `.wasp` file is the same name that will be used in the `name` column of pg-boss tables. If you change a name that had a `schedule` associated with it, pg-boss will continue scheduling those jobs but they will have no handlers associated, and will thus become stale and expire. To resolve this, you can remove the applicable row from the `schedule` table in the `pgboss` schema of your database.
* If you remove a `schedule` from a job, you will need to do the above as well.
* If you wish to deploy to Heroku, you need to set an additional environment variable called `PG_BOSS_NEW_OPTIONS` to `{"connectionString":"<REGULAR_HEROKU_DATABASE_URL>","ssl":{"rejectUnauthorized":false}}`. This is because pg-boss uses the `pg` extension, which does not seem to connect to Heroku over SSL by default, which Heroku requires. Additionally, Heroku uses a self-signed cert, so we must handle that as well.
* [https://devcenter.heroku.com/articles/connecting-heroku-postgres#connecting-in-node-js](https://devcenter.heroku.com/articles/connecting-heroku-postgres#connecting-in-node-js)
* `perform: dict` required
* `fn: ExtImport` required
* An `async` function that performs the work. Since Wasp executes Jobs on the server, the import path must lead to a NodeJS file.
* It receives the following arguments:
* `args: Input`: The data passed to the job when it's submitted.
* `context: { entities: Entities }`: The context object containing any declared entities.
Here's an example of a `perform.fn` function:
* JavaScript
* TypeScript
src/workers/bar.js
```
export const foo = async ({ name }, context) => { console.log(`Hello ${name}!`) const tasks = await context.entities.Task.findMany({}) return { tasks }}
```
* `executorOptions: dict`
Executor-specific default options to use when submitting jobs. These are passed directly through and you should consult the documentation for the job executor. These can be overridden during invocation with `submit()` or in a `schedule`.
* `pgBoss: JSON`
See the docs for [pg-boss](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#sendname-data-options).
* `schedule: dict`
* `cron: string` required
A 5-placeholder format cron expression string. See rationale for minute-level precision [here](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#scheduling).
_If you need help building cron expressions, Check out_ _[Crontab guru](https://crontab.guru/#0_*_*_*_*)._
* `args: JSON`
The arguments to pass to the `perform.fn` function when invoked.
* `executorOptions: dict`
Executor-specific options to use when submitting jobs. These are passed directly through and you should consult the documentation for the job executor. The `perform.executorOptions` are the default options, and `schedule.executorOptions` can override/extend those.
* `pgBoss: JSON`
See the docs for [pg-boss](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#sendname-data-options).
* `entities: [Entity]`
A list of entities you wish to use inside your Job (similar to [Queries and Actions](https://wasp-lang.dev/docs/data-model/operations/queries#using-entities-in-queries)).
### JavaScript API[](#javascript-api "Direct link to JavaScript API")
* Importing a Job:
* JavaScript
* TypeScript
someAction.js
```
import { mySpecialJob } from 'wasp/server/jobs'
```
* `submit(jobArgs, executorOptions)`
* `jobArgs: Input`
* `executorOptions: object`
Submits a Job to be executed by an executor, optionally passing in a JSON job argument your job handler function receives, and executor-specific submit options.
* JavaScript
* TypeScript
someAction.js
```
const submittedJob = await mySpecialJob.submit({ job: "args" })
```
* `delay(startAfter)`
* `startAfter: int | string | Date` required
Delaying the invocation of the job handler. The delay can be one of:
* Integer: number of seconds to delay. \[Default 0\]
* String: ISO date string to run at.
* Date: Date to run at.
* JavaScript
* TypeScript
someAction.js
```
const submittedJob = await mySpecialJob .delay(10) .submit({ job: "args" }, { "retryLimit": 2 })
```
#### Tracking[](#tracking "Direct link to Tracking")
The return value of `submit()` is an instance of `SubmittedJob`, which has the following fields:
* `jobId`: The ID for the job in that executor.
* `jobName`: The name of the job you used in your `.wasp` file.
* `executorName`: The Symbol of the name of the job executor.
There are also some namespaced, job executor-specific objects.
* For pg-boss, you may access: `pgBoss`
* `details()`: pg-boss specific job detail information. [Reference](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#getjobbyidid)
* `cancel()`: attempts to cancel a job. [Reference](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#cancelid)
* `resume()`: attempts to resume a canceled job. [Reference](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#resumeid) | null | https://wasp-lang.dev/docs/advanced/jobs | In most web apps, users send requests to the server and receive responses with some data. When the server responds quickly, the app feels responsive and smooth. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece4bed483ae8-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:53 GMT | Tue, 23 Apr 2024 15:24:53 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=Yz7MxJNx4eTNJv3cVrRrMvQR7CrrmWdqexmNXqSUPMCbtIca97XvpXfL7MCU3DyMCn%2B%2FvmSzVyOmWAydY5jnfPiFJWQ9EYGyHr6Zjy3IRZePZwSPr5EsIWvIn4WJ3EJM"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 1da8f97a5d544887b5a041a831c91b8d61624142 | null | 4F36:3FA5BA:381475F:4330A61:6627D06C | null | MISS | cache-iad-kiad7000070-IAD | S1713885293.460107,VS0,VE61 | null | null | en | og:image | https://wasp-lang.dev/docs/advanced/jobs | og:url | Recurring Jobs | Wasp | og:title | In most web apps, users send requests to the server and receive responses with some data. When the server responds quickly, the app feels responsive and smooth. | og:description | Recurring Jobs | Wasp | null | Recurring Jobs
In most web apps, users send requests to the server and receive responses with some data. When the server responds quickly, the app feels responsive and smooth.
What if the server needs extra time to fully process the request? This might mean sending an email or making a slow HTTP request to an external API. In that case, it's a good idea to respond to the user as soon as possible and do the remaining work in the background.
Wasp supports background jobs that can help you with this:
Jobs persist between server restarts,
Jobs can be retried if they fail,
Jobs can be delayed until a future time,
Jobs can have a recurring schedule.
Using Jobs
Job Definition and Usage
Let's write an example Job that will print a message to the console and return a list of tasks from the database.
Start by creating a Job declaration in your .wasp file:
JavaScript
TypeScript
main.wasp
job mySpecialJob {
executor: PgBoss,
perform: {
fn: import { foo } from "@src/workers/bar"
},
entities: [Task],
}
After declaring the Job, implement its worker function:
JavaScript
TypeScript
src/workers/bar.js
export const foo = async ({ name }, context) => {
console.log(`Hello ${name}!`)
const tasks = await context.entities.Task.findMany({})
return { tasks }
}
The worker function
The worker function must be an async function. The function's return value represents the Job's result.
The worker function accepts two arguments:
args: The data passed into the job when it's submitted.
context: { entities }: The context object containing entities you put in the Job declaration.
After successfully defining the job, you can submit work to be done in your Operations or setupFn (or any other NodeJS code):
JavaScript
TypeScript
someAction.js
import { mySpecialJob } from 'wasp/server/jobs'
const submittedJob = await mySpecialJob.submit({ job: "Johnny" })
// Or, if you'd prefer it to execute in the future, just add a .delay().
// It takes a number of seconds, Date, or ISO date string.
await mySpecialJob
.delay(10)
.submit({ name: "Johnny" })
And that's it. Your job will be executed by PgBoss as if you called foo({ name: "Johnny" }).
In our example, foo takes an argument, but passing arguments to jobs is not a requirement. It depends on how you've implemented your worker function.
Recurring Jobs
If you have work that needs to be done on some recurring basis, you can add a schedule to your job declaration:
JavaScript
TypeScript
main.wasp
job mySpecialJob {
executor: PgBoss,
perform: {
fn: import { foo } from "@src/workers/bar"
},
schedule: {
cron: "0 * * * *",
args: {=json { "job": "args" } json=} // optional
}
}
In this example, you don't need to invoke anything in . You can imagine foo({ job: "args" }) getting automatically scheduled and invoked for you every hour.
API Reference
Declaring Jobs
JavaScript
TypeScript
main.wasp
job mySpecialJob {
executor: PgBoss,
perform: {
fn: import { foo } from "@src/workers/bar",
executorOptions: {
pgBoss: {=json { "retryLimit": 1 } json=}
}
},
schedule: {
cron: "*/5 * * * *",
args: {=json { "foo": "bar" } json=},
executorOptions: {
pgBoss: {=json { "retryLimit": 0 } json=}
}
},
entities: [Task],
}
The Job declaration has the following fields:
executor: JobExecutor required
Job executors
Our jobs need job executors to handle the scheduling, monitoring, and execution.
PgBoss is currently our only job executor, and is recommended for low-volume production use cases. It requires your app.db.system to be PostgreSQL.
We have selected pg-boss as our first job executor to handle the low-volume, basic job queue workloads many web applications have. By using PostgreSQL (and SKIP LOCKED) as its storage and synchronization mechanism, it allows us to provide many job queue pros without any additional infrastructure or complex management.
info
Keep in mind that pg-boss jobs run alongside your other server-side code, so they are not appropriate for CPU-heavy workloads. Additionally, some care is required if you modify scheduled jobs. Please see pg-boss details below for more information.
pg-boss details
pg-boss provides many useful features, which can be found here.
When you add pg-boss to a Wasp project, it will automatically add a new schema to your database called pgboss with some internal tracking tables, including job and schedule. pg-boss tables have a name column in most tables that will correspond to your Job identifier. Additionally, these tables maintain arguments, states, return values, retry information, start and expiration times, and other metadata required by pg-boss.
If you need to customize the creation of the pg-boss instance, you can set an environment variable called PG_BOSS_NEW_OPTIONS to a stringified JSON object containing these initialization parameters. NOTE: Setting this overwrites all Wasp defaults, so you must include database connection information as well.
pg-boss considerations
Wasp starts pg-boss alongside your web server's application, where both are simultaneously operational. This means that jobs running via pg-boss and the rest of the server logic (like Operations) share the CPU, therefore you should avoid running CPU-intensive tasks via jobs.
Wasp does not (yet) support independent, horizontal scaling of pg-boss-only applications, nor starting them as separate workers/processes/threads.
The job name/identifier in your .wasp file is the same name that will be used in the name column of pg-boss tables. If you change a name that had a schedule associated with it, pg-boss will continue scheduling those jobs but they will have no handlers associated, and will thus become stale and expire. To resolve this, you can remove the applicable row from the schedule table in the pgboss schema of your database.
If you remove a schedule from a job, you will need to do the above as well.
If you wish to deploy to Heroku, you need to set an additional environment variable called PG_BOSS_NEW_OPTIONS to {"connectionString":"<REGULAR_HEROKU_DATABASE_URL>","ssl":{"rejectUnauthorized":false}}. This is because pg-boss uses the pg extension, which does not seem to connect to Heroku over SSL by default, which Heroku requires. Additionally, Heroku uses a self-signed cert, so we must handle that as well.
https://devcenter.heroku.com/articles/connecting-heroku-postgres#connecting-in-node-js
perform: dict required
fn: ExtImport required
An async function that performs the work. Since Wasp executes Jobs on the server, the import path must lead to a NodeJS file.
It receives the following arguments:
args: Input: The data passed to the job when it's submitted.
context: { entities: Entities }: The context object containing any declared entities.
Here's an example of a perform.fn function:
JavaScript
TypeScript
src/workers/bar.js
export const foo = async ({ name }, context) => {
console.log(`Hello ${name}!`)
const tasks = await context.entities.Task.findMany({})
return { tasks }
}
executorOptions: dict
Executor-specific default options to use when submitting jobs. These are passed directly through and you should consult the documentation for the job executor. These can be overridden during invocation with submit() or in a schedule.
pgBoss: JSON
See the docs for pg-boss.
schedule: dict
cron: string required
A 5-placeholder format cron expression string. See rationale for minute-level precision here.
If you need help building cron expressions, Check out Crontab guru.
args: JSON
The arguments to pass to the perform.fn function when invoked.
executorOptions: dict
Executor-specific options to use when submitting jobs. These are passed directly through and you should consult the documentation for the job executor. The perform.executorOptions are the default options, and schedule.executorOptions can override/extend those.
pgBoss: JSON
See the docs for pg-boss.
entities: [Entity]
A list of entities you wish to use inside your Job (similar to Queries and Actions).
JavaScript API
Importing a Job:
JavaScript
TypeScript
someAction.js
import { mySpecialJob } from 'wasp/server/jobs'
submit(jobArgs, executorOptions)
jobArgs: Input
executorOptions: object
Submits a Job to be executed by an executor, optionally passing in a JSON job argument your job handler function receives, and executor-specific submit options.
JavaScript
TypeScript
someAction.js
const submittedJob = await mySpecialJob.submit({ job: "args" })
delay(startAfter)
startAfter: int | string | Date required
Delaying the invocation of the job handler. The delay can be one of:
Integer: number of seconds to delay. [Default 0]
String: ISO date string to run at.
Date: Date to run at.
JavaScript
TypeScript
someAction.js
const submittedJob = await mySpecialJob
.delay(10)
.submit({ job: "args" }, { "retryLimit": 2 })
Tracking
The return value of submit() is an instance of SubmittedJob, which has the following fields:
jobId: The ID for the job in that executor.
jobName: The name of the job you used in your .wasp file.
executorName: The Symbol of the name of the job executor.
There are also some namespaced, job executor-specific objects.
For pg-boss, you may access: pgBoss
details(): pg-boss specific job detail information. Reference
cancel(): attempts to cancel a job. Reference
resume(): attempts to resume a canceled job. Reference | https://wasp-lang.dev/docs/advanced/jobs |
|
1 | null | 2024-04-23T15:15:01.020Z | https://wasp-lang.dev/docs/advanced/links | https://wasp-lang.dev/docs | http | Version: 0.13.0
## Type-Safe Links
If you are using Typescript, you can use Wasp's custom `Link` component to create type-safe links to other pages on your site.
## Using the `Link` Component[](#using-the-link-component "Direct link to using-the-link-component")
After you defined a route:
main.wasp
```
route TaskRoute { path: "/task/:id", to: TaskPage }page TaskPage { ... }
```
You can get the benefits of type-safe links by using the `Link` component from `wasp/client/router`:
TaskList.tsx
```
import { Link } from 'wasp/client/router'export const TaskList = () => { // ... return ( <div> {tasks.map((task) => ( <Link key={task.id} to="/task/:id" {/* 👆 You must provide a valid path here */} params={{ id: task.id }}> {/* 👆 All the params must be correctly passed in */} {task.description} </Link> ))} </div> )}
```
### Using Search Query & Hash[](#using-search-query--hash "Direct link to Using Search Query & Hash")
You can also pass `search` and `hash` props to the `Link` component:
TaskList.tsx
```
<Link to="/task/:id" params={{ id: task.id }} search={{ sortBy: 'date' }} hash="comments"> {task.description}</Link>
```
This will result in a link like this: `/task/1?sortBy=date#comments`. Check out the [API Reference](#link-component) for more details.
## The `routes` Object[](#the-routes-object "Direct link to the-routes-object")
You can also get all the pages in your app with the `routes` object:
TaskList.tsx
```
import { routes } from 'wasp/client/router'const linkToTask = routes.TaskRoute.build({ params: { id: 1 } })
```
This will result in a link like this: `/task/1`.
You can also pass `search` and `hash` props to the `build` function. Check out the [API Reference](#routes-object) for more details.
## API Reference[](#api-reference "Direct link to API Reference")
### `Link` Component[](#link-component "Direct link to link-component")
The `Link` component accepts the following props:
* `to` required
* A valid Wasp Route path from your `main.wasp` file.
* `params: { [name: string]: string | number }` required (if the path contains params)
* An object with keys and values for each param in the path.
* For example, if the path is `/task/:id`, then the `params` prop must be `{ id: 1 }`. Wasp supports required and optional params.
* `search: string[][] | Record<string, string> | string | URLSearchParams`
* Any valid input for `URLSearchParams` constructor.
* For example, the object `{ sortBy: 'date' }` becomes `?sortBy=date`.
* `hash: string`
* all other props that the `react-router-dom`'s [Link](https://v5.reactrouter.com/web/api/Link) component accepts
### `routes` Object[](#routes-object "Direct link to routes-object")
The `routes` object contains a function for each route in your app.
router.tsx
```
export const routes = { // RootRoute has a path like "/" RootRoute: { build: (options?: { search?: string[][] | Record<string, string> | string | URLSearchParams hash?: string }) => // ... }, // DetailRoute has a path like "/task/:id/:something?" DetailRoute: { build: ( options: { params: { id: ParamValue; something?: ParamValue; }, search?: string[][] | Record<string, string> | string | URLSearchParams hash?: string } ) => // ... }}
```
The `params` object is required if the route contains params. The `search` and `hash` parameters are optional.
You can use the `routes` object like this:
```
import { routes } from 'wasp/client/router'const linkToRoot = routes.RootRoute.build()const linkToTask = routes.DetailRoute.build({ params: { id: 1 } })
``` | null | https://wasp-lang.dev/docs/advanced/links | If you are using Typescript, you can use Wasp's custom Link component to create type-safe links to other pages on your site. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece7a3f841ff8-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:00 GMT | Tue, 23 Apr 2024 15:25:00 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=MAP%2Fx7eioSuN6z4hlx%2FwYa%2BdvSERe0fnfZaqJlP9Hg0uVWx67%2FqOnK8unpmg0XWI6J2j%2FaVd3JErpLu98lgDnCRVrajWe1hJVlkZ3pMtl9tL554nmIl%2BFtU3ZzoTT5D8"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | e4e5ac828080433b81c9c99b8a88319dff1f0b86 | null | 7372:111A3A:3AEA7BC:45CB80A:6627D074 | HIT | MISS | cache-iad-kiad7000074-IAD | S1713885301.878157,VS0,VE10 | null | null | en | og:image | https://wasp-lang.dev/docs/advanced/links | og:url | Type-Safe Links | Wasp | og:title | If you are using Typescript, you can use Wasp's custom Link component to create type-safe links to other pages on your site. | og:description | Type-Safe Links | Wasp | null | Version: 0.13.0
Type-Safe Links
If you are using Typescript, you can use Wasp's custom Link component to create type-safe links to other pages on your site.
Using the Link Component
After you defined a route:
main.wasp
route TaskRoute { path: "/task/:id", to: TaskPage }
page TaskPage { ... }
You can get the benefits of type-safe links by using the Link component from wasp/client/router:
TaskList.tsx
import { Link } from 'wasp/client/router'
export const TaskList = () => {
// ...
return (
<div>
{tasks.map((task) => (
<Link
key={task.id}
to="/task/:id"
{/* 👆 You must provide a valid path here */}
params={{ id: task.id }}>
{/* 👆 All the params must be correctly passed in */}
{task.description}
</Link>
))}
</div>
)
}
Using Search Query & Hash
You can also pass search and hash props to the Link component:
TaskList.tsx
<Link
to="/task/:id"
params={{ id: task.id }}
search={{ sortBy: 'date' }}
hash="comments"
>
{task.description}
</Link>
This will result in a link like this: /task/1?sortBy=date#comments. Check out the API Reference for more details.
The routes Object
You can also get all the pages in your app with the routes object:
TaskList.tsx
import { routes } from 'wasp/client/router'
const linkToTask = routes.TaskRoute.build({ params: { id: 1 } })
This will result in a link like this: /task/1.
You can also pass search and hash props to the build function. Check out the API Reference for more details.
API Reference
Link Component
The Link component accepts the following props:
to required
A valid Wasp Route path from your main.wasp file.
params: { [name: string]: string | number } required (if the path contains params)
An object with keys and values for each param in the path.
For example, if the path is /task/:id, then the params prop must be { id: 1 }. Wasp supports required and optional params.
search: string[][] | Record<string, string> | string | URLSearchParams
Any valid input for URLSearchParams constructor.
For example, the object { sortBy: 'date' } becomes ?sortBy=date.
hash: string
all other props that the react-router-dom's Link component accepts
routes Object
The routes object contains a function for each route in your app.
router.tsx
export const routes = {
// RootRoute has a path like "/"
RootRoute: {
build: (options?: {
search?: string[][] | Record<string, string> | string | URLSearchParams
hash?: string
}) => // ...
},
// DetailRoute has a path like "/task/:id/:something?"
DetailRoute: {
build: (
options: {
params: { id: ParamValue; something?: ParamValue; },
search?: string[][] | Record<string, string> | string | URLSearchParams
hash?: string
}
) => // ...
}
}
The params object is required if the route contains params. The search and hash parameters are optional.
You can use the routes object like this:
import { routes } from 'wasp/client/router'
const linkToRoot = routes.RootRoute.build()
const linkToTask = routes.DetailRoute.build({ params: { id: 1 } }) | https://wasp-lang.dev/docs/advanced/links |
|
1 | null | 2024-04-23T15:15:00.924Z | https://wasp-lang.dev/docs/advanced/middleware-config | https://wasp-lang.dev/docs | http | Version: 0.13.0
## Configuring Middleware
Wasp comes with a minimal set of useful Express middleware in every application. While this is good for most users, we realize some may wish to add, modify, or remove some of these choices both globally, or on a per-`api`/path basis.
## Default Global Middleware 🌍[](#default-global-middleware- "Direct link to Default Global Middleware 🌍")
Wasp's Express server has the following middleware by default:
* [Helmet](https://helmetjs.github.io/): Helmet helps you secure your Express apps by setting various HTTP headers. _It's not a silver bullet, but it's a good start._
* [CORS](https://github.com/expressjs/cors#readme): CORS is a package for providing a middleware that can be used to enable [CORS](https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS) with various options.
note
CORS middleware is required for the frontend to communicate with the backend.
* [Morgan](https://github.com/expressjs/morgan#readme): HTTP request logger middleware.
* [express.json](https://expressjs.com/en/api.html#express.json) (which uses [body-parser](https://github.com/expressjs/body-parser#bodyparserjsonoptions)): parses incoming request bodies in a middleware before your handlers, making the result available under the `req.body` property.
note
JSON middleware is required for [Operations](https://wasp-lang.dev/docs/data-model/operations/overview) to function properly.
* [express.urlencoded](https://expressjs.com/en/api.html#express.urlencoded) (which uses [body-parser](https://expressjs.com/en/resources/middleware/body-parser.html#bodyparserurlencodedoptions)): returns middleware that only parses urlencoded bodies and only looks at requests where the `Content-Type` header matches the type option.
* [cookieParser](https://github.com/expressjs/cookie-parser#readme): parses Cookie header and populates `req.cookies` with an object keyed by the cookie names.
## Customization[](#customization "Direct link to Customization")
You have three places where you can customize middleware:
1. [global](#1-customize-global-middleware): here, any changes will apply by default _to all operations (`query` and `action`) and `api`._ This is helpful if you wanted to add support for multiple domains to CORS, for example.
Modifying global middleware
Please treat modifications to global middleware with extreme care as they will affect all operations and APIs. If you are unsure, use one of the other two options.
2. [per-api](#2-customize-api-specific-middleware): you can override middleware for a specific api route (e.g. `POST /webhook/callback`). This is helpful if you want to disable JSON parsing for some callback, for example.
3. [per-path](#3-customize-per-path-middleware): this is helpful if you need to customize middleware for all methods under a given path.
* It's helpful for things like "complex CORS requests" which may need to apply to both `OPTIONS` and `GET`, or to apply some middleware to a _set of `api` routes_.
### Default Middleware Definitions[](#default-middleware-definitions "Direct link to Default Middleware Definitions")
Below is the actual definitions of default middleware which you can override.
* JavaScript
* TypeScript
```
const defaultGlobalMiddleware = new Map([ ['helmet', helmet()], ['cors', cors({ origin: config.allowedCORSOrigins })], ['logger', logger('dev')], ['express.json', express.json()], ['express.urlencoded', express.urlencoded({ extended: false })], ['cookieParser', cookieParser()]])
```
## 1\. Customize Global Middleware[](#1-customize-global-middleware "Direct link to 1. Customize Global Middleware")
If you would like to modify the middleware for _all_ operations and APIs, you can do something like:
* JavaScript
* TypeScript
main.wasp
```
app todoApp { // ... server: { setupFn: import setup from "@src/serverSetup", middlewareConfigFn: import { serverMiddlewareFn } from "@src/serverSetup" },}
```
src/serverSetup.js
```
import cors from 'cors'import { config } from 'wasp/server'export const serverMiddlewareFn = (middlewareConfig) => { // Example of adding extra domains to CORS. middlewareConfig.set('cors', cors({ origin: [config.frontendUrl, 'https://example1.com', 'https://example2.com'] })) return middlewareConfig}
```
## 2\. Customize `api`\-specific Middleware[](#2-customize-api-specific-middleware "Direct link to 2-customize-api-specific-middleware")
If you would like to modify the middleware for a single API, you can do something like:
* JavaScript
* TypeScript
main.wasp
```
// ...api webhookCallback { fn: import { webhookCallback } from "@src/apis", middlewareConfigFn: import { webhookCallbackMiddlewareFn } from "@src/apis", httpRoute: (POST, "/webhook/callback"), auth: false}
```
src/apis.js
```
import express from 'express'export const webhookCallback = (req, res, _context) => { res.json({ msg: req.body.length })}export const webhookCallbackMiddlewareFn = (middlewareConfig) => { console.log('webhookCallbackMiddlewareFn: Swap express.json for express.raw') middlewareConfig.delete('express.json') middlewareConfig.set('express.raw', express.raw({ type: '*/*' })) return middlewareConfig}
```
note
This gets installed on a per-method basis. Behind the scenes, this results in code like:
```
router.post('/webhook/callback', webhookCallbackMiddleware, ...)
```
## 3\. Customize Per-Path Middleware[](#3-customize-per-path-middleware "Direct link to 3. Customize Per-Path Middleware")
If you would like to modify the middleware for all API routes under some common path, you can define a `middlewareConfigFn` on an `apiNamespace`:
* JavaScript
* TypeScript
main.wasp
```
// ...apiNamespace fooBar { middlewareConfigFn: import { fooBarNamespaceMiddlewareFn } from "@src/apis", path: "/foo/bar"}
```
src/apis.js
```
export const fooBarNamespaceMiddlewareFn = (middlewareConfig) => { const customMiddleware = (_req, _res, next) => { console.log('fooBarNamespaceMiddlewareFn: custom middleware') next() } middlewareConfig.set('custom.middleware', customMiddleware) return middlewareConfig}
```
note
This gets installed at the router level for the path. Behind the scenes, this results in something like:
```
router.use('/foo/bar', fooBarNamespaceMiddleware)
``` | null | https://wasp-lang.dev/docs/advanced/middleware-config | Wasp comes with a minimal set of useful Express middleware in every application. While this is good for most users, we realize some may wish to add, modify, or remove some of these choices both globally, or on a per-api/path basis. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece79fad9593d-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:00 GMT | Tue, 23 Apr 2024 15:25:00 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=wpO2jkXKCeXzfvcRMTjNFbeJrvr%2FbsIMmOVRJ8yt6FCg%2FrtDYVKS8KyFh7rJJyi%2F0j1kiFl2WhPbqKGAKo5F%2B%2BgACgVlYaX%2BWyUrI9qwspgPj1VjwP3SKEHDSmBrp4oB"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 035bf635442e0b7ea459448f4607c7a841a3d807 | null | B518:2C5630:3C5573A:475B1A2:6627D074 | HIT | MISS | cache-iad-kiad7000141-IAD | S1713885301.820875,VS0,VE14 | null | null | en | og:image | https://wasp-lang.dev/docs/advanced/middleware-config | og:url | Configuring Middleware | Wasp | og:title | Wasp comes with a minimal set of useful Express middleware in every application. While this is good for most users, we realize some may wish to add, modify, or remove some of these choices both globally, or on a per-api/path basis. | og:description | Configuring Middleware | Wasp | null | Version: 0.13.0
Configuring Middleware
Wasp comes with a minimal set of useful Express middleware in every application. While this is good for most users, we realize some may wish to add, modify, or remove some of these choices both globally, or on a per-api/path basis.
Default Global Middleware 🌍
Wasp's Express server has the following middleware by default:
Helmet: Helmet helps you secure your Express apps by setting various HTTP headers. It's not a silver bullet, but it's a good start.
CORS: CORS is a package for providing a middleware that can be used to enable CORS with various options.
note
CORS middleware is required for the frontend to communicate with the backend.
Morgan: HTTP request logger middleware.
express.json (which uses body-parser): parses incoming request bodies in a middleware before your handlers, making the result available under the req.body property.
note
JSON middleware is required for Operations to function properly.
express.urlencoded (which uses body-parser): returns middleware that only parses urlencoded bodies and only looks at requests where the Content-Type header matches the type option.
cookieParser: parses Cookie header and populates req.cookies with an object keyed by the cookie names.
Customization
You have three places where you can customize middleware:
global: here, any changes will apply by default to all operations (query and action) and api. This is helpful if you wanted to add support for multiple domains to CORS, for example.
Modifying global middleware
Please treat modifications to global middleware with extreme care as they will affect all operations and APIs. If you are unsure, use one of the other two options.
per-api: you can override middleware for a specific api route (e.g. POST /webhook/callback). This is helpful if you want to disable JSON parsing for some callback, for example.
per-path: this is helpful if you need to customize middleware for all methods under a given path.
It's helpful for things like "complex CORS requests" which may need to apply to both OPTIONS and GET, or to apply some middleware to a set of api routes.
Default Middleware Definitions
Below is the actual definitions of default middleware which you can override.
JavaScript
TypeScript
const defaultGlobalMiddleware = new Map([
['helmet', helmet()],
['cors', cors({ origin: config.allowedCORSOrigins })],
['logger', logger('dev')],
['express.json', express.json()],
['express.urlencoded', express.urlencoded({ extended: false })],
['cookieParser', cookieParser()]
])
1. Customize Global Middleware
If you would like to modify the middleware for all operations and APIs, you can do something like:
JavaScript
TypeScript
main.wasp
app todoApp {
// ...
server: {
setupFn: import setup from "@src/serverSetup",
middlewareConfigFn: import { serverMiddlewareFn } from "@src/serverSetup"
},
}
src/serverSetup.js
import cors from 'cors'
import { config } from 'wasp/server'
export const serverMiddlewareFn = (middlewareConfig) => {
// Example of adding extra domains to CORS.
middlewareConfig.set('cors', cors({ origin: [config.frontendUrl, 'https://example1.com', 'https://example2.com'] }))
return middlewareConfig
}
2. Customize api-specific Middleware
If you would like to modify the middleware for a single API, you can do something like:
JavaScript
TypeScript
main.wasp
// ...
api webhookCallback {
fn: import { webhookCallback } from "@src/apis",
middlewareConfigFn: import { webhookCallbackMiddlewareFn } from "@src/apis",
httpRoute: (POST, "/webhook/callback"),
auth: false
}
src/apis.js
import express from 'express'
export const webhookCallback = (req, res, _context) => {
res.json({ msg: req.body.length })
}
export const webhookCallbackMiddlewareFn = (middlewareConfig) => {
console.log('webhookCallbackMiddlewareFn: Swap express.json for express.raw')
middlewareConfig.delete('express.json')
middlewareConfig.set('express.raw', express.raw({ type: '*/*' }))
return middlewareConfig
}
note
This gets installed on a per-method basis. Behind the scenes, this results in code like:
router.post('/webhook/callback', webhookCallbackMiddleware, ...)
3. Customize Per-Path Middleware
If you would like to modify the middleware for all API routes under some common path, you can define a middlewareConfigFn on an apiNamespace:
JavaScript
TypeScript
main.wasp
// ...
apiNamespace fooBar {
middlewareConfigFn: import { fooBarNamespaceMiddlewareFn } from "@src/apis",
path: "/foo/bar"
}
src/apis.js
export const fooBarNamespaceMiddlewareFn = (middlewareConfig) => {
const customMiddleware = (_req, _res, next) => {
console.log('fooBarNamespaceMiddlewareFn: custom middleware')
next()
}
middlewareConfig.set('custom.middleware', customMiddleware)
return middlewareConfig
}
note
This gets installed at the router level for the path. Behind the scenes, this results in something like:
router.use('/foo/bar', fooBarNamespaceMiddleware) | https://wasp-lang.dev/docs/advanced/middleware-config |
|
1 | null | 2024-04-23T15:15:04.916Z | https://wasp-lang.dev/docs/general/cli | https://wasp-lang.dev/docs | http | ## CLI Reference
This guide provides an overview of the Wasp CLI commands, arguments, and options.
## Overview[](#overview "Direct link to Overview")
Once [installed](https://wasp-lang.dev/docs/quick-start), you can use the wasp command from your command line.
If you run the `wasp` command without any arguments, it will show you a list of available commands and their descriptions:
```
USAGE wasp <command> [command-args]COMMANDS GENERAL new [<name>] [args] Creates a new Wasp project. Run it without arguments for interactive mode. OPTIONS: -t|--template <template-name> Check out the templates list here: https://github.com/wasp-lang/starters new:ai <app-name> <app-description> [<config-json>] Uses AI to create a new Wasp project just based on the app name and the description. You can do the same thing with `wasp new` interactively. Run `wasp new:ai` for more info. version Prints current version of CLI. waspls Run Wasp Language Server. Add --help to get more info. completion Prints help on bash completion. uninstall Removes Wasp from your system. IN PROJECT start Runs Wasp app in development mode, watching for file changes. start db Starts managed development database for you. db <db-cmd> [args] Executes a database command. Run 'wasp db' for more info. clean Deletes all generated code, all cached artifacts, and the node_modules dir. Wasp equivalent of 'have you tried closing and opening it again?'. build Generates full web app code, ready for deployment. Use when deploying or ejecting. deploy Deploys your Wasp app to cloud hosting providers. telemetry Prints telemetry status. deps Prints the dependencies that Wasp uses in your project. dockerfile Prints the contents of the Wasp generated Dockerfile. info Prints basic information about the current Wasp project. test Executes tests in your project. studio (experimental) GUI for inspecting your Wasp app.EXAMPLES wasp new MyApp wasp start wasp db migrate-devDocs: https://wasp-lang.dev/docsDiscord (chat): https://discord.gg/rzdnErXNewsletter: https://wasp-lang.dev/#signup
```
## Commands[](#commands "Direct link to Commands")
### Creating a New Project[](#creating-a-new-project "Direct link to Creating a New Project")
* Use `wasp new` to start the interactive mode for setting up a new Wasp project.
This will prompt you to input the project name and to select a template. The chosen template will then be used to generate the project directory with the specified name.
```
$ wasp newEnter the project name (e.g. my-project) ▸ MyFirstProjectChoose a starter template[1] basic (default) Simple starter template with a single page.[2] todo-ts Simple but well-rounded Wasp app implemented with Typescript & full-stack type safety.[3] saas Everything a SaaS needs! Comes with Auth, ChatGPT API, Tailwind, Stripe payments and more. Check out https://opensaas.sh/ for more details.[4] embeddings Comes with code for generating vector embeddings and performing vector similarity search.[5] ai-generated 🤖 Describe an app in a couple of sentences and have Wasp AI generate initial code for you. (experimental)▸ 1🐝 --- Creating your project from the "basic" template... -------------------------Created new Wasp app in ./MyFirstProject directory!To run your new app, do: cd MyFirstProject wasp db start
```
* To skip the interactive mode and create a new Wasp project with the default template, use `wasp new <project-name>`.
```
$ wasp new MyFirstProject🐝 --- Creating your project from the "basic" template... -------------------------Created new Wasp app in ./MyFirstProject directory!To run your new app, do: cd MyFirstProject wasp db start
```
### Project Commands[](#project-commands "Direct link to Project Commands")
* `wasp start` launches the Wasp app in development mode. It automatically opens a browser tab with your application running and watches for any changes to .wasp or files in `src/` to automatically reflect in the browser. It also shows messages from the web app, the server and the database on stdout/stderr.
* `wasp start db` starts the database for you. This can be very handy since you don't need to spin up your own database or provide its connection URL to the Wasp app.
* `wasp clean` removes all generated code and other cached artifacts. If using SQlite, it also deletes the SQlite database. Think of this as the Wasp version of the classic "turn it off and on again" solution.
```
$ wasp clean🐝 --- Deleting the .wasp/ directory... -------------------------------------------✅ --- Deleted the .wasp/ directory. ----------------------------------------------🐝 --- Deleting the node_modules/ directory... ------------------------------------✅ --- Deleted the node_modules/ directory. ---------------------------------------
```
* `wasp build` generates the complete web app code, which is ready for [deployment](https://wasp-lang.dev/docs/advanced/deployment/overview). Use this command when you're deploying or ejecting. The generated code is stored in the `.wasp/build` folder.
* `wasp deploy` makes it easy to get your app hosted on the web.
Currently, Wasp offers support for [Fly.io](https://fly.io/). If you prefer a different hosting provider, feel free to let us know on Discord or submit a PR by updating [this TypeScript app](https://github.com/wasp-lang/wasp/tree/main/waspc/packages/deploy).
Read more about automatic deployment [here](https://wasp-lang.dev/docs/advanced/deployment/cli).
* `wasp telemetry` displays the status of [telemetry](https://wasp-lang.dev/docs/telemetry).
```
$ wasp telemetryTelemetry is currently: ENABLEDTelemetry cache directory: /home/user/.cache/wasp/telemetry/Last time telemetry data was sent for this project: 2021-05-27 09:21:16.79537226 UTCOur telemetry is anonymized and very limited in its scope: check https://wasp-lang.dev/docs/telemetry for more details.
```
* `wasp deps` lists the dependencies that Wasp uses in your project.
* `wasp info` provides basic details about the current Wasp project.
* `wasp studio` shows you an graphical overview of your application in a graph: pages, queries, actions, data model etc.
### Database Commands[](#database-commands "Direct link to Database Commands")
Wasp provides a suite of commands for managing the database. These commands all begin with `db` and primarily execute Prisma commands behind the scenes.
* `wasp db migrate-dev` synchronizes the development database with the current state of the schema (entities). If there are any changes in the schema, it generates a new migration and applies any pending migrations to the database.
* The `--name foo` option allows you to specify a name for the migration, while the `--create-only` option lets you create an empty migration without applying it.
* `wasp db studio` opens the GUI for inspecting your database.
### Bash Completion[](#bash-completion "Direct link to Bash Completion")
To set up Bash completion, run the `wasp completion` command and follow the instructions.
### Miscellaneous Commands[](#miscellaneous-commands "Direct link to Miscellaneous Commands")
* `wasp version` displays the current version of the CLI.
```
$ wasp version0.12.0If you wish to install/switch to the latest version of Wasp, do:curl -sSL https://get.wasp-lang.dev/installer.sh | sh -sIf you want specific x.y.z version of Wasp, do:curl -sSL https://get.wasp-lang.dev/installer.sh | sh -s -- -v x.y.zCheck https://github.com/wasp-lang/wasp/releases for the list of valid versions, including the latest one.
```
* `wasp uninstall` removes Wasp from your system.
```
$ wasp uninstall🐝 --- Uninstalling Wasp ... ------------------------------------------------------ We will remove the following directories: {home}/.local/share/wasp-lang/ {home}/.cache/wasp/ We will also remove the following files: {home}/.local/bin/wasp Are you sure you want to continue? [y/N] y ✅ --- Uninstalled Wasp -----------------------------------------------------------
``` | null | https://wasp-lang.dev/docs/general/cli | This guide provides an overview of the Wasp CLI commands, arguments, and options. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece932b4a6f9b-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:04 GMT | Tue, 23 Apr 2024 15:25:04 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=hTSPj6fIi%2FjX4Sz53bJsCWPBHah6rvkbcPN8Cr5k7FPffzKPDavjLsBkAOAgQatSEQkyYu4BoZ7z8qavwGwPx5XcBNZhUh0%2BqmZxCHDBBY%2FxeiZRniHNCqtL9t1oG2XW"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | b54aaa17aacabd5b5af7cde36a5e40646551c81b | null | 944E:173D:DA27B:10A009:6627D078 | HIT | MISS | cache-iad-kiad7000163-IAD | S1713885305.851893,VS0,VE10 | null | null | en | og:image | https://wasp-lang.dev/docs/general/cli | og:url | CLI Reference | Wasp | og:title | This guide provides an overview of the Wasp CLI commands, arguments, and options. | og:description | CLI Reference | Wasp | null | CLI Reference
This guide provides an overview of the Wasp CLI commands, arguments, and options.
Overview
Once installed, you can use the wasp command from your command line.
If you run the wasp command without any arguments, it will show you a list of available commands and their descriptions:
USAGE
wasp <command> [command-args]
COMMANDS
GENERAL
new [<name>] [args] Creates a new Wasp project. Run it without arguments for interactive mode.
OPTIONS:
-t|--template <template-name>
Check out the templates list here: https://github.com/wasp-lang/starters
new:ai <app-name> <app-description> [<config-json>]
Uses AI to create a new Wasp project just based on the app name and the description.
You can do the same thing with `wasp new` interactively.
Run `wasp new:ai` for more info.
version Prints current version of CLI.
waspls Run Wasp Language Server. Add --help to get more info.
completion Prints help on bash completion.
uninstall Removes Wasp from your system.
IN PROJECT
start Runs Wasp app in development mode, watching for file changes.
start db Starts managed development database for you.
db <db-cmd> [args] Executes a database command. Run 'wasp db' for more info.
clean Deletes all generated code, all cached artifacts, and the node_modules dir.
Wasp equivalent of 'have you tried closing and opening it again?'.
build Generates full web app code, ready for deployment. Use when deploying or ejecting.
deploy Deploys your Wasp app to cloud hosting providers.
telemetry Prints telemetry status.
deps Prints the dependencies that Wasp uses in your project.
dockerfile Prints the contents of the Wasp generated Dockerfile.
info Prints basic information about the current Wasp project.
test Executes tests in your project.
studio (experimental) GUI for inspecting your Wasp app.
EXAMPLES
wasp new MyApp
wasp start
wasp db migrate-dev
Docs: https://wasp-lang.dev/docs
Discord (chat): https://discord.gg/rzdnErX
Newsletter: https://wasp-lang.dev/#signup
Commands
Creating a New Project
Use wasp new to start the interactive mode for setting up a new Wasp project.
This will prompt you to input the project name and to select a template. The chosen template will then be used to generate the project directory with the specified name.
$ wasp new
Enter the project name (e.g. my-project) ▸ MyFirstProject
Choose a starter template
[1] basic (default)
Simple starter template with a single page.
[2] todo-ts
Simple but well-rounded Wasp app implemented with Typescript & full-stack type safety.
[3] saas
Everything a SaaS needs! Comes with Auth, ChatGPT API, Tailwind, Stripe payments and more. Check out https://opensaas.sh/ for more details.
[4] embeddings
Comes with code for generating vector embeddings and performing vector similarity search.
[5] ai-generated
🤖 Describe an app in a couple of sentences and have Wasp AI generate initial code for you. (experimental)
▸ 1
🐝 --- Creating your project from the "basic" template... -------------------------
Created new Wasp app in ./MyFirstProject directory!
To run your new app, do:
cd MyFirstProject
wasp db start
To skip the interactive mode and create a new Wasp project with the default template, use wasp new <project-name>.
$ wasp new MyFirstProject
🐝 --- Creating your project from the "basic" template... -------------------------
Created new Wasp app in ./MyFirstProject directory!
To run your new app, do:
cd MyFirstProject
wasp db start
Project Commands
wasp start launches the Wasp app in development mode. It automatically opens a browser tab with your application running and watches for any changes to .wasp or files in src/ to automatically reflect in the browser. It also shows messages from the web app, the server and the database on stdout/stderr.
wasp start db starts the database for you. This can be very handy since you don't need to spin up your own database or provide its connection URL to the Wasp app.
wasp clean removes all generated code and other cached artifacts. If using SQlite, it also deletes the SQlite database. Think of this as the Wasp version of the classic "turn it off and on again" solution.
$ wasp clean
🐝 --- Deleting the .wasp/ directory... -------------------------------------------
✅ --- Deleted the .wasp/ directory. ----------------------------------------------
🐝 --- Deleting the node_modules/ directory... ------------------------------------
✅ --- Deleted the node_modules/ directory. ---------------------------------------
wasp build generates the complete web app code, which is ready for deployment. Use this command when you're deploying or ejecting. The generated code is stored in the .wasp/build folder.
wasp deploy makes it easy to get your app hosted on the web.
Currently, Wasp offers support for Fly.io. If you prefer a different hosting provider, feel free to let us know on Discord or submit a PR by updating this TypeScript app.
Read more about automatic deployment here.
wasp telemetry displays the status of telemetry.
$ wasp telemetry
Telemetry is currently: ENABLED
Telemetry cache directory: /home/user/.cache/wasp/telemetry/
Last time telemetry data was sent for this project: 2021-05-27 09:21:16.79537226 UTC
Our telemetry is anonymized and very limited in its scope: check https://wasp-lang.dev/docs/telemetry for more details.
wasp deps lists the dependencies that Wasp uses in your project.
wasp info provides basic details about the current Wasp project.
wasp studio shows you an graphical overview of your application in a graph: pages, queries, actions, data model etc.
Database Commands
Wasp provides a suite of commands for managing the database. These commands all begin with db and primarily execute Prisma commands behind the scenes.
wasp db migrate-dev synchronizes the development database with the current state of the schema (entities). If there are any changes in the schema, it generates a new migration and applies any pending migrations to the database.
The --name foo option allows you to specify a name for the migration, while the --create-only option lets you create an empty migration without applying it.
wasp db studio opens the GUI for inspecting your database.
Bash Completion
To set up Bash completion, run the wasp completion command and follow the instructions.
Miscellaneous Commands
wasp version displays the current version of the CLI.
$ wasp version
0.12.0
If you wish to install/switch to the latest version of Wasp, do:
curl -sSL https://get.wasp-lang.dev/installer.sh | sh -s
If you want specific x.y.z version of Wasp, do:
curl -sSL https://get.wasp-lang.dev/installer.sh | sh -s -- -v x.y.z
Check https://github.com/wasp-lang/wasp/releases for the list of valid versions, including the latest one.
wasp uninstall removes Wasp from your system.
$ wasp uninstall
🐝 --- Uninstalling Wasp ... ------------------------------------------------------
We will remove the following directories:
{home}/.local/share/wasp-lang/
{home}/.cache/wasp/
We will also remove the following files:
{home}/.local/bin/wasp
Are you sure you want to continue? [y/N]
y
✅ --- Uninstalled Wasp ----------------------------------------------------------- | https://wasp-lang.dev/docs/general/cli |
|
2 | null | 2024-04-23T15:15:04.814Z | https://wasp-lang.dev/docs/0.12.0/vision | https://wasp-lang.dev/docs/vision | http | ## Vision
With Wasp, we want to make developing web apps easy and enjoyable, for novices and experts in web development alike.
Ideal we are striving for is that programming in Wasp feels like describing an app using a human language - like writing a specification document where you describe primarily your requirements and as little implementation details as possible. Creating a new production-ready web app should be easy and deploying it to production should be straightforward.
That is why we believe Wasp needs to be a programming language (DSL) and not a library - we want to capture all parts of the web app into one integrated system that is perfectly tailored just for that purpose.
On the other hand, we believe that trying to capture every single detail in one language would not be reasonable. There are solutions out there that work very well for the specific task they aim to solve (React for web components, CSS/HTML for design/markup, JS/TS for logic, ...) and we don't want to replace them with Wasp. Instead, we see Wasp as a declarative "glue" code uniting all these specific solutions and providing a higher-level notion of the web app above them.
Wasp is still early in its development and therefore far from where we imagine it will be in the future. This is what we imagine:
* **Declarative, static language** with simple basic rules and **that understands a lot of web app concepts** - "horizontal language". Supports multiple files/modules, libraries.
* **Integrates seamlessly with the most popular technologies** for building specific, more complex parts of the web app (React, CSS, JS, ...). They can be used inline (mixed with Wasp code) or provided via external files.
* **Has hatches (escape mechanisms) that allow you to customize your web app** in all the right places, but remain hidden until you need them.
* **Entity (data model) is a first-class citizen** - defined via custom Wasp syntax and it integrates very closely with the rest of the features, serving as one of the central concepts around which everything is built.
* **Out of the box** support for CRUD UI based on the Entities, to get you quickly going, but also customizable to some level.
* **"Smart" operations (queries and actions)** that in most cases automatically figure out when to update, and if not it is easy to define custom logic to compensate for that. User worries about client-server gap as little as possible.
* Support, directly in Wasp, for **declaratively defining simple components and operations**.
* Besides Wasp as a programming language, there will also be a **visual builder that generates/edits Wasp code**, allowing non-developers to participate in development. Since Wasp is declarative, we imagine such builder to naturally follow from Wasp language.
* **Server side rendering, caching, packaging, security**, ... -> all those are taken care of by Wasp. You tell Wasp what you want, and Wasp figures out how to do it.
* As **simple deployment to production/staging** as it gets.
* While it comes with the official implementation(s), **Wasp language will not be coupled with the single implementation**. Others can provide implementations that compile to different web app stacks. | null | https://wasp-lang.dev/docs/0.12.0/vision | With Wasp, we want to make developing web apps easy and enjoyable, for novices and experts in web development alike. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece92acc020cf-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:04 GMT | Tue, 23 Apr 2024 15:25:04 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=AgYKbY8hKH879Cp12ILF14ffFH6KqzlUHeH1g%2FIWL1pzDpusLTUAN7LHPORBo9I%2Bi3xRbkWMNxtLgw1ufSp%2BDHHQFOtDiHu1KxIv6x0f7YBse8SLY6Ms6wZEhHVtAxtU"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 3cd339f5ab1830793f31581a94a50c4e0d3e08e7 | null | 4664:209F3A:3A804E3:4586DD3:6627D078 | HIT | MISS | cache-iad-kiad7000086-IAD | S1713885305.772350,VS0,VE22 | null | null | en | og:image | https://wasp-lang.dev/docs/0.12.0/vision | og:url | Vision | Wasp | og:title | With Wasp, we want to make developing web apps easy and enjoyable, for novices and experts in web development alike. | og:description | Vision | Wasp | null | Vision
With Wasp, we want to make developing web apps easy and enjoyable, for novices and experts in web development alike.
Ideal we are striving for is that programming in Wasp feels like describing an app using a human language - like writing a specification document where you describe primarily your requirements and as little implementation details as possible. Creating a new production-ready web app should be easy and deploying it to production should be straightforward.
That is why we believe Wasp needs to be a programming language (DSL) and not a library - we want to capture all parts of the web app into one integrated system that is perfectly tailored just for that purpose.
On the other hand, we believe that trying to capture every single detail in one language would not be reasonable. There are solutions out there that work very well for the specific task they aim to solve (React for web components, CSS/HTML for design/markup, JS/TS for logic, ...) and we don't want to replace them with Wasp. Instead, we see Wasp as a declarative "glue" code uniting all these specific solutions and providing a higher-level notion of the web app above them.
Wasp is still early in its development and therefore far from where we imagine it will be in the future. This is what we imagine:
Declarative, static language with simple basic rules and that understands a lot of web app concepts - "horizontal language". Supports multiple files/modules, libraries.
Integrates seamlessly with the most popular technologies for building specific, more complex parts of the web app (React, CSS, JS, ...). They can be used inline (mixed with Wasp code) or provided via external files.
Has hatches (escape mechanisms) that allow you to customize your web app in all the right places, but remain hidden until you need them.
Entity (data model) is a first-class citizen - defined via custom Wasp syntax and it integrates very closely with the rest of the features, serving as one of the central concepts around which everything is built.
Out of the box support for CRUD UI based on the Entities, to get you quickly going, but also customizable to some level.
"Smart" operations (queries and actions) that in most cases automatically figure out when to update, and if not it is easy to define custom logic to compensate for that. User worries about client-server gap as little as possible.
Support, directly in Wasp, for declaratively defining simple components and operations.
Besides Wasp as a programming language, there will also be a visual builder that generates/edits Wasp code, allowing non-developers to participate in development. Since Wasp is declarative, we imagine such builder to naturally follow from Wasp language.
Server side rendering, caching, packaging, security, ... -> all those are taken care of by Wasp. You tell Wasp what you want, and Wasp figures out how to do it.
As simple deployment to production/staging as it gets.
While it comes with the official implementation(s), Wasp language will not be coupled with the single implementation. Others can provide implementations that compile to different web app stacks. | https://wasp-lang.dev/docs/0.12.0/vision |
|
1 | null | 2024-04-23T15:15:04.580Z | https://wasp-lang.dev/docs/general/language | https://wasp-lang.dev/docs | http | ## Wasp Language (.wasp)
Wasp language (what you write in .wasp files) is a declarative, statically typed, domain-specific language (DSL).
It is a quite simple language, closer to JSON, CSS or SQL than to e.g. Javascript or Python, since it is not a general programming language, but more of a configuration language.
It is pretty intuitive to learn (there isn't much to learn really!) and you can probably do just fine without reading this page and learning from the rest of the docs as you go, but if you want a bit more formal definition and deeper understanding of how it works, then read on!
## Declarations[](#declarations "Direct link to Declarations")
The central point of Wasp language are **declarations**, and Wasp code is at the end just a bunch of declarations, each of them describing a part of your web app.
```
app MyApp { title: "My app"}route RootRoute { path: "/", to: DashboardPage }page DashboardPage { component: import { DashboardPage } from "@src/Dashboard.jsx"}
```
In the example above we described a web app via three declarations: `app MyApp { ... }`, `route RootRoute { ... }` and `page DashboardPage { ... }`.
Syntax for writing a declaration is `<declaration_type> <declaration_name> <declaration_body>`, where:
* `<declaration_type>` is one of the declaration types offered by Wasp (`app`, `route`, ...)
* `<declaration_name>` is an identifier chosen by you to name this specific declaration
* `<declaration_body>` is the value/definition of the declaration itself, which has to match the specific declaration body type expected by the chosen declaration type.
So, for `app` declaration above, we have:
* declaration type `app`
* declaration name `MyApp` (we could have used any other identifier, like `foobar`, `foo_bar`, or `hi3Ho`)
* declaration body `{ title: "My app" }`, which is a dictionary with field `title` that has string value. Type of this dictionary is in line with the declaration body type of the `app` declaration type. If we provided something else, e.g. changed `title` to `little`, we would get a type error from Wasp compiler since that does not match the expected type of the declaration body for `app`.
Each declaration has a meaning behind it that describes how your web app should behave and function.
All the other types in Wasp language (primitive types (`string`, `number`), composite types (`dict`, `list`), enum types (`DbSystem`), ...) are used to define the declaration bodies.
## Complete List of Wasp Types[](#complete-list-of-wasp-types "Direct link to Complete List of Wasp Types")
Wasp's type system can be divided into two main categories of types: **fundamental types** and **domain types**.
While fundamental types are here to be basic building blocks of a language and are very similar to what you would see in other popular languages, domain types are what make Wasp special, as they model the concepts of a web app like `page`, `route` and similar.
* Fundamental types ([source of truth](https://github.com/wasp-lang/wasp/blob/main/waspc/src/Wasp/Analyzer/Type.hs))
* Primitive types
* **string** (`"foo"`, `"they said: \"hi\""`)
* **bool** (`true`, `false`)
* **number** (`12`, `14.5`)
* **declaration reference** (name of existing declaration: `TaskPage`, `updateTask`)
* **ExtImport** (external import) (`import Foo from "@src/bar.js"`, `import { Smth } from "@src/a/b.js"`)
* The path has to start with "@src". The rest is relative to the `src` directory.
* Import has to be a default import `import Foo` or a single named import `import { Foo }`.
* **json** (`{=json { a: 5, b: ["hi"] } json=}`)
* **psl** (Prisma Schema Language) (`{=psl <psl data model syntax> psl=}`)
* Check [Prisma docs](https://www.prisma.io/docs/concepts/components/prisma-schema/data-model) for the syntax of psl data model.
* Composite types
* **dict** (dictionary) (`{ a: 5, b: "foo" }`)
* **list** (`[1, 2, 3]`)
* **tuple** (`(1, "bar")`, `(2, 4, true)`)
* Tuples can be of size 2, 3 and 4.
* Domain types ([source of truth](https://github.com/wasp-lang/wasp/blob/main/waspc/src/Wasp/Analyzer/StdTypeDefinitions.hs))
* Declaration types
* **action**
* **api**
* **apiNamespace**
* **app**
* **entity**
* **job**
* **page**
* **query**
* **route**
* **crud**
* Enum types
* **DbSystem**
* **HttpMethod**
* **JobExecutor**
* **EmailProvider**
You can find more details about each of the domain types, both regarding their body types and what they mean, in the corresponding doc pages covering their features. | null | https://wasp-lang.dev/docs/general/language | Wasp language (what you write in .wasp files) is a declarative, statically typed, domain-specific language (DSL). | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece842a9f0824-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:02 GMT | Tue, 23 Apr 2024 14:20:39 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=3aEX4GbcTdK%2BVV1XR0GFYuPn3MUOAbvnWsAUPkVD0uShl6wBVWtIi%2Fn8e%2BOyoBaxGDg%2F%2B7wmRB4eg%2FBxqpsmNaxrU4OtzBMzlvS1pK3Q5TzC0D1ZeHYzgeYxx2OmexuJ"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | HIT | 0 | ab5a11dd402cfe72e65e128d8a25062fcc5648ef | null | F6E8:53E77:39D71A9:44D8405:6627C15E | null | MISS | cache-iad-kiad7000175-IAD | S1713885302.459545,VS0,VE7 | null | null | en | og:image | https://wasp-lang.dev/docs/general/language | og:url | Wasp Language (.wasp) | Wasp | og:title | Wasp language (what you write in .wasp files) is a declarative, statically typed, domain-specific language (DSL). | og:description | Wasp Language (.wasp) | Wasp | null | Wasp Language (.wasp)
Wasp language (what you write in .wasp files) is a declarative, statically typed, domain-specific language (DSL).
It is a quite simple language, closer to JSON, CSS or SQL than to e.g. Javascript or Python, since it is not a general programming language, but more of a configuration language.
It is pretty intuitive to learn (there isn't much to learn really!) and you can probably do just fine without reading this page and learning from the rest of the docs as you go, but if you want a bit more formal definition and deeper understanding of how it works, then read on!
Declarations
The central point of Wasp language are declarations, and Wasp code is at the end just a bunch of declarations, each of them describing a part of your web app.
app MyApp {
title: "My app"
}
route RootRoute { path: "/", to: DashboardPage }
page DashboardPage {
component: import { DashboardPage } from "@src/Dashboard.jsx"
}
In the example above we described a web app via three declarations: app MyApp { ... }, route RootRoute { ... } and page DashboardPage { ... }.
Syntax for writing a declaration is <declaration_type> <declaration_name> <declaration_body>, where:
<declaration_type> is one of the declaration types offered by Wasp (app, route, ...)
<declaration_name> is an identifier chosen by you to name this specific declaration
<declaration_body> is the value/definition of the declaration itself, which has to match the specific declaration body type expected by the chosen declaration type.
So, for app declaration above, we have:
declaration type app
declaration name MyApp (we could have used any other identifier, like foobar, foo_bar, or hi3Ho)
declaration body { title: "My app" }, which is a dictionary with field title that has string value. Type of this dictionary is in line with the declaration body type of the app declaration type. If we provided something else, e.g. changed title to little, we would get a type error from Wasp compiler since that does not match the expected type of the declaration body for app.
Each declaration has a meaning behind it that describes how your web app should behave and function.
All the other types in Wasp language (primitive types (string, number), composite types (dict, list), enum types (DbSystem), ...) are used to define the declaration bodies.
Complete List of Wasp Types
Wasp's type system can be divided into two main categories of types: fundamental types and domain types.
While fundamental types are here to be basic building blocks of a language and are very similar to what you would see in other popular languages, domain types are what make Wasp special, as they model the concepts of a web app like page, route and similar.
Fundamental types (source of truth)
Primitive types
string ("foo", "they said: \"hi\"")
bool (true, false)
number (12, 14.5)
declaration reference (name of existing declaration: TaskPage, updateTask)
ExtImport (external import) (import Foo from "@src/bar.js", import { Smth } from "@src/a/b.js")
The path has to start with "@src". The rest is relative to the src directory.
Import has to be a default import import Foo or a single named import import { Foo }.
json ({=json { a: 5, b: ["hi"] } json=})
psl (Prisma Schema Language) ({=psl <psl data model syntax> psl=})
Check Prisma docs for the syntax of psl data model.
Composite types
dict (dictionary) ({ a: 5, b: "foo" })
list ([1, 2, 3])
tuple ((1, "bar"), (2, 4, true))
Tuples can be of size 2, 3 and 4.
Domain types (source of truth)
Declaration types
action
api
apiNamespace
app
entity
job
page
query
route
crud
Enum types
DbSystem
HttpMethod
JobExecutor
EmailProvider
You can find more details about each of the domain types, both regarding their body types and what they mean, in the corresponding doc pages covering their features. | https://wasp-lang.dev/docs/general/language |
|
1 | 200 | 2024-04-23T15:14:57.869Z | https://wasp-lang.dev/docs/advanced/web-sockets | https://wasp-lang.dev/docs | browser | ## Web Sockets
Wasp provides a fully integrated WebSocket experience by utilizing [Socket.IO](https://socket.io/) on the client and server.
We handle making sure your URLs are correctly setup, CORS is enabled, and provide a useful `useSocket` and `useSocketListener` abstractions for use in React components.
To get started, you need to:
1. Define your WebSocket logic on the server.
2. Enable WebSockets in your Wasp file, and connect it with your server logic.
3. Use WebSockets on the client, in React, via `useSocket` and `useSocketListener`.
4. Optionally, type the WebSocket events and payloads for full-stack type safety.
Let's go through setting up WebSockets step by step, starting with enabling WebSockets in your Wasp file.
## Turn On WebSockets in Your Wasp File[](#turn-on-websockets-in-your-wasp-file "Direct link to Turn On WebSockets in Your Wasp File")
We specify that we are using WebSockets by adding `webSocket` to our `app` and providing the required `fn`. You can optionally change the auto-connect behavior.
* JavaScript
* TypeScript
todoApp.wasp
```
app todoApp { // ... webSocket: { fn: import { webSocketFn } from "@src/webSocket", autoConnect: true, // optional, default: true },}
```
## Defining the Events Handler[](#defining-the-events-handler "Direct link to Defining the Events Handler")
Let's define the WebSockets server with all of the events and handler functions.
### `webSocketFn` Function[](#websocketfn-function "Direct link to websocketfn-function")
On the server, you will get Socket.IO `io: Server` argument and `context` for your WebSocket function. The `context` object give you access to all of the entities from your Wasp app.
You can use this `io` object to register callbacks for all the regular [Socket.IO events](https://socket.io/docs/v4/server-api/). Also, if a user is logged in, you will have a `socket.data.user` on the server.
This is how we can define our `webSocketFn` function:
* JavaScript
* TypeScript
src/webSocket.js
```
import { v4 as uuidv4 } from 'uuid'import { getFirstProviderUserId } from 'wasp/auth'export const webSocketFn = (io, context) => { io.on('connection', (socket) => { const username = getFirstProviderUserId(socket.data.user) ?? 'Unknown' console.log('a user connected: ', username) socket.on('chatMessage', async (msg) => { console.log('message: ', msg) io.emit('chatMessage', { id: uuidv4(), username, text: msg }) // You can also use your entities here: // await context.entities.SomeEntity.create({ someField: msg }) }) })}
```
## Using the WebSocket On The Client[](#using-the-websocket-on-the-client "Direct link to Using the WebSocket On The Client")
### `useSocket` Hook[](#usesocket-hook "Direct link to usesocket-hook")
Client access to WebSockets is provided by the `useSocket` hook. It returns:
* `socket: Socket` for sending and receiving events.
* `isConnected: boolean` for showing a display of the Socket.IO connection status.
* Note: Wasp automatically connects and establishes a WebSocket connection from the client to the server by default, so you do not need to explicitly `socket.connect()` or `socket.disconnect()`.
* If you set `autoConnect: false` in your Wasp file, then you should call these as needed.
All components using `useSocket` share the same underlying `socket`.
### `useSocketListener` Hook[](#usesocketlistener-hook "Direct link to usesocketlistener-hook")
Additionally, there is a `useSocketListener: (event, callback) => void` hook which is used for registering event handlers. It takes care of unregistering the handler on unmount.
* JavaScript
* TypeScript
src/ChatPage.jsx
```
import React, { useState } from 'react'import { useSocket, useSocketListener,} from 'wasp/client/webSocket'export const ChatPage = () => { const [messageText, setMessageText] = useState('') const [messages, setMessages] = useState([]) const { socket, isConnected } = useSocket() useSocketListener('chatMessage', logMessage) function logMessage(msg) { setMessages((priorMessages) => [msg, ...priorMessages]) } function handleSubmit(e) { e.preventDefault() socket.emit('chatMessage', messageText) setMessageText('') } const messageList = messages.map((msg) => ( <li key={msg.id}> <em>{msg.username}</em>: {msg.text} </li> )) const connectionIcon = isConnected ? '🟢' : '🔴' return ( <> <h2>Chat {connectionIcon}</h2> <div> <form onSubmit={handleSubmit}> <div> <div> <input type="text" value={messageText} onChange={(e) => setMessageText(e.target.value)} /> </div> <div> <button type="submit">Submit</button> </div> </div> </form> <ul>{messageList}</ul> </div> </> )}
```
## API Reference[](#api-reference "Direct link to API Reference")
* JavaScript
* TypeScript
todoApp.wasp
```
app todoApp { // ... webSocket: { fn: import { webSocketFn } from "@src/webSocket", autoConnect: true, // optional, default: true },}
```
The `webSocket` dict has the following fields:
* `fn: WebSocketFn` required
The function that defines the WebSocket events and handlers.
* `autoConnect: bool`
Whether to automatically connect to the WebSocket server. Default: `true`. | null | https://wasp-lang.dev/docs/advanced/web-sockets | Wasp provides a fully integrated WebSocket experience by utilizing Socket.IO on the client and server. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece4be84c4bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:14:53 GMT | Tue, 23 Apr 2024 15:24:53 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=o8YWNfAahZDNiHO1VLz5GtPcpoHbh9TFiSAfgge2qEMVzJtLen3GXThQF99VU5LeG%2BL34dsUZoT34M66942nDd0oOih0ys5P9adCum5kaB%2BpPdJhkRnsQlIOAqVZzi3L"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | baf8ddd98722ed8902ec7ca93c7118f8e00af371 | h2 | FA84:1766:541CD3:64C534:6627D06D | HIT | MISS | cache-nyc-kteb1890087-NYC | S1713885293.432420, VS0, VE92 | null | null | en | og:image | https://wasp-lang.dev/docs/advanced/web-sockets | og:url | Web Sockets | Wasp | og:title | Wasp provides a fully integrated WebSocket experience by utilizing Socket.IO on the client and server. | og:description | Web Sockets | Wasp | null | Web Sockets
Wasp provides a fully integrated WebSocket experience by utilizing Socket.IO on the client and server.
We handle making sure your URLs are correctly setup, CORS is enabled, and provide a useful useSocket and useSocketListener abstractions for use in React components.
To get started, you need to:
Define your WebSocket logic on the server.
Enable WebSockets in your Wasp file, and connect it with your server logic.
Use WebSockets on the client, in React, via useSocket and useSocketListener.
Optionally, type the WebSocket events and payloads for full-stack type safety.
Let's go through setting up WebSockets step by step, starting with enabling WebSockets in your Wasp file.
Turn On WebSockets in Your Wasp File
We specify that we are using WebSockets by adding webSocket to our app and providing the required fn. You can optionally change the auto-connect behavior.
JavaScript
TypeScript
todoApp.wasp
app todoApp {
// ...
webSocket: {
fn: import { webSocketFn } from "@src/webSocket",
autoConnect: true, // optional, default: true
},
}
Defining the Events Handler
Let's define the WebSockets server with all of the events and handler functions.
webSocketFn Function
On the server, you will get Socket.IO io: Server argument and context for your WebSocket function. The context object give you access to all of the entities from your Wasp app.
You can use this io object to register callbacks for all the regular Socket.IO events. Also, if a user is logged in, you will have a socket.data.user on the server.
This is how we can define our webSocketFn function:
JavaScript
TypeScript
src/webSocket.js
import { v4 as uuidv4 } from 'uuid'
import { getFirstProviderUserId } from 'wasp/auth'
export const webSocketFn = (io, context) => {
io.on('connection', (socket) => {
const username = getFirstProviderUserId(socket.data.user) ?? 'Unknown'
console.log('a user connected: ', username)
socket.on('chatMessage', async (msg) => {
console.log('message: ', msg)
io.emit('chatMessage', { id: uuidv4(), username, text: msg })
// You can also use your entities here:
// await context.entities.SomeEntity.create({ someField: msg })
})
})
}
Using the WebSocket On The Client
useSocket Hook
Client access to WebSockets is provided by the useSocket hook. It returns:
socket: Socket for sending and receiving events.
isConnected: boolean for showing a display of the Socket.IO connection status.
Note: Wasp automatically connects and establishes a WebSocket connection from the client to the server by default, so you do not need to explicitly socket.connect() or socket.disconnect().
If you set autoConnect: false in your Wasp file, then you should call these as needed.
All components using useSocket share the same underlying socket.
useSocketListener Hook
Additionally, there is a useSocketListener: (event, callback) => void hook which is used for registering event handlers. It takes care of unregistering the handler on unmount.
JavaScript
TypeScript
src/ChatPage.jsx
import React, { useState } from 'react'
import {
useSocket,
useSocketListener,
} from 'wasp/client/webSocket'
export const ChatPage = () => {
const [messageText, setMessageText] = useState('')
const [messages, setMessages] = useState([])
const { socket, isConnected } = useSocket()
useSocketListener('chatMessage', logMessage)
function logMessage(msg) {
setMessages((priorMessages) => [msg, ...priorMessages])
}
function handleSubmit(e) {
e.preventDefault()
socket.emit('chatMessage', messageText)
setMessageText('')
}
const messageList = messages.map((msg) => (
<li key={msg.id}>
<em>{msg.username}</em>: {msg.text}
</li>
))
const connectionIcon = isConnected ? '🟢' : '🔴'
return (
<>
<h2>Chat {connectionIcon}</h2>
<div>
<form onSubmit={handleSubmit}>
<div>
<div>
<input
type="text"
value={messageText}
onChange={(e) => setMessageText(e.target.value)}
/>
</div>
<div>
<button type="submit">Submit</button>
</div>
</div>
</form>
<ul>{messageList}</ul>
</div>
</>
)
}
API Reference
JavaScript
TypeScript
todoApp.wasp
app todoApp {
// ...
webSocket: {
fn: import { webSocketFn } from "@src/webSocket",
autoConnect: true, // optional, default: true
},
}
The webSocket dict has the following fields:
fn: WebSocketFn required
The function that defines the WebSocket events and handlers.
autoConnect: bool
Whether to automatically connect to the WebSocket server. Default: true. | https://wasp-lang.dev/docs/advanced/web-sockets |
|
1 | 200 | 2024-04-23T15:15:05.751Z | https://wasp-lang.dev/docs/advanced/apis | https://wasp-lang.dev/docs | browser | ## Custom HTTP API Endpoints
In Wasp, the default client-server interaction mechanism is through [Operations](https://wasp-lang.dev/docs/data-model/operations/overview). However, if you need a specific URL method/path, or a specific response, Operations may not be suitable for you. For these cases, you can use an `api`. Best of all, they should look and feel very familiar.
## How to Create an API[](#how-to-create-an-api "Direct link to How to Create an API")
APIs are used to tie a JS function to a certain endpoint e.g. `POST /something/special`. They are distinct from Operations and have no client-side helpers (like `useQuery`).
To create a Wasp API, you must:
1. Declare the API in Wasp using the `api` declaration
2. Define the API's NodeJS implementation
After completing these two steps, you'll be able to call the API from the client code (via our `Axios` wrapper), or from the outside world.
### Declaring the API in Wasp[](#declaring-the-api-in-wasp "Direct link to Declaring the API in Wasp")
First, we need to declare the API in the Wasp file and you can easily do this with the `api` declaration:
* JavaScript
* TypeScript
main.wasp
```
// ...api fooBar { // APIs and their implementations don't need to (but can) have the same name. fn: import { fooBar } from "@src/apis", httpRoute: (GET, "/foo/bar")}
```
Read more about the supported fields in the [API Reference](#api-reference).
### Defining the API's NodeJS Implementation[](#defining-the-apis-nodejs-implementation "Direct link to Defining the API's NodeJS Implementation")
After you defined the API, it should be implemented as a NodeJS function that takes three arguments:
1. `req`: Express Request object
2. `res`: Express Response object
3. `context`: An additional context object **injected into the API by Wasp**. This object contains user session information, as well as information about entities. The examples here won't use the context for simplicity purposes. You can read more about it in the [section about using entities in APIs](#using-entities-in-apis).
* JavaScript
* TypeScript
src/apis.js
```
export const fooBar = (req, res, context) => { res.set("Access-Control-Allow-Origin", "*"); // Example of modifying headers to override Wasp default CORS middleware. res.json({ msg: `Hello, ${context.user ? "registered user" : "stranger"}!` });};
```
## Using the API[](#using-the-api "Direct link to Using the API")
### Using the API externally[](#using-the-api-externally "Direct link to Using the API externally")
To use the API externally, you simply call the endpoint using the method and path you used.
For example, if your app is running at `https://example.com` then from the above you could issue a `GET` to `https://example/com/foo/callback` (in your browser, Postman, `curl`, another web service, etc.).
### Using the API from the Client[](#using-the-api-from-the-client "Direct link to Using the API from the Client")
To use the API from your client, including with auth support, you can import the Axios wrapper from `wasp/client/api` and invoke a call. For example:
* JavaScript
* TypeScript
src/pages/SomePage.jsx
```
import React, { useEffect } from "react";import { api } from "wasp/client/api";async function fetchCustomRoute() { const res = await api.get("/foo/bar"); console.log(res.data);}export const Foo = () => { useEffect(() => { fetchCustomRoute(); }, []); return <>// ...</>;};
```
#### Making Sure CORS Works[](#making-sure-cors-works "Direct link to Making Sure CORS Works")
APIs are designed to be as flexible as possible, hence they don't utilize the default middleware like Operations do. As a result, to use these APIs on the client side, you must ensure that CORS (Cross-Origin Resource Sharing) is enabled.
You can do this by defining custom middleware for your APIs in the Wasp file.
* JavaScript
* TypeScript
For example, an `apiNamespace` is a simple declaration used to apply some `middlewareConfigFn` to all APIs under some specific path:
main.wasp
```
apiNamespace fooBar { middlewareConfigFn: import { fooBarNamespaceMiddlewareFn } from "@src/apis", path: "/foo"}
```
And then in the implementation file:
src/apis.js
```
export const apiMiddleware = (config) => { return config;};
```
We are returning the default middleware which enables CORS for all APIs under the `/foo` path.
For more information about middleware configuration, please see: [Middleware Configuration](https://wasp-lang.dev/docs/advanced/middleware-config)
## Using Entities in APIs[](#using-entities-in-apis "Direct link to Using Entities in APIs")
In many cases, resources used in APIs will be [Entities](https://wasp-lang.dev/docs/data-model/entities). To use an Entity in your API, add it to the `api` declaration in Wasp:
* JavaScript
* TypeScript
main.wasp
```
api fooBar { fn: import { fooBar } from "@src/apis", entities: [Task], httpRoute: (GET, "/foo/bar")}
```
Wasp will inject the specified Entity into the APIs `context` argument, giving you access to the Entity's Prisma API:
* JavaScript
* TypeScript
src/apis.js
```
export const fooBar = (req, res, context) => { res.json({ count: await context.entities.Task.count() });};
```
The object `context.entities.Task` exposes `prisma.task` from [Prisma's CRUD API](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-client/crud).
## API Reference[](#api-reference "Direct link to API Reference")
* JavaScript
* TypeScript
main.wasp
```
api fooBar { fn: import { fooBar } from "@src/apis", httpRoute: (GET, "/foo/bar"), entities: [Task], auth: true, middlewareConfigFn: import { apiMiddleware } from "@src/apis"}
```
The `api` declaration has the following fields:
* `fn: ExtImport` required
The import statement of the APIs NodeJs implementation.
* `httpRoute: (HttpMethod, string)` required
The HTTP (method, path) pair, where the method can be one of:
* `ALL`, `GET`, `POST`, `PUT` or `DELETE`
* and path is an Express path `string`.
* `entities: [Entity]`
A list of entities you wish to use inside your API. You can read more about it [here](#using-entities-in-apis).
* `auth: bool`
If auth is enabled, this will default to `true` and provide a `context.user` object. If you do not wish to attempt to parse the JWT in the Authorization Header, you should set this to `false`.
* `middlewareConfigFn: ExtImport`
The import statement to an Express middleware config function for this API. See more in [middleware section](https://wasp-lang.dev/docs/advanced/middleware-config) of the docs. | null | https://wasp-lang.dev/docs/advanced/apis | In Wasp, the default client-server interaction mechanism is through Operations. However, if you need a specific URL method/path, or a specific response, Operations may not be suitable for you. For these cases, you can use an api. Best of all, they should look and feel very familiar. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ece7e9af24bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:01 GMT | Tue, 23 Apr 2024 15:25:01 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=dehS5cK7AwlDbJxxF6r3%2F9Z8JHmrZhGemZlba9i27XL2lZ8E2mPl1nb23c0hfSVPyvtk%2BZlI8sQnLOY7LCDnz%2BlEsdAXo%2BL4NzO7ZeADZKEwlWRwmRkMyyledQCBhdGi"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | fba35c602079b55e9549eeff68d4d4c26ab837e2 | h2 | 9D32:354B3:3A336F8:454FCA3:6627D073 | HIT | MISS | cache-nyc-kteb1890087-NYC | S1713885302.548840, VS0, VE109 | null | null | en | og:image | https://wasp-lang.dev/docs/advanced/apis | og:url | Custom HTTP API Endpoints | Wasp | og:title | In Wasp, the default client-server interaction mechanism is through Operations. However, if you need a specific URL method/path, or a specific response, Operations may not be suitable for you. For these cases, you can use an api. Best of all, they should look and feel very familiar. | og:description | Custom HTTP API Endpoints | Wasp | null | Custom HTTP API Endpoints
In Wasp, the default client-server interaction mechanism is through Operations. However, if you need a specific URL method/path, or a specific response, Operations may not be suitable for you. For these cases, you can use an api. Best of all, they should look and feel very familiar.
How to Create an API
APIs are used to tie a JS function to a certain endpoint e.g. POST /something/special. They are distinct from Operations and have no client-side helpers (like useQuery).
To create a Wasp API, you must:
Declare the API in Wasp using the api declaration
Define the API's NodeJS implementation
After completing these two steps, you'll be able to call the API from the client code (via our Axios wrapper), or from the outside world.
Declaring the API in Wasp
First, we need to declare the API in the Wasp file and you can easily do this with the api declaration:
JavaScript
TypeScript
main.wasp
// ...
api fooBar { // APIs and their implementations don't need to (but can) have the same name.
fn: import { fooBar } from "@src/apis",
httpRoute: (GET, "/foo/bar")
}
Read more about the supported fields in the API Reference.
Defining the API's NodeJS Implementation
After you defined the API, it should be implemented as a NodeJS function that takes three arguments:
req: Express Request object
res: Express Response object
context: An additional context object injected into the API by Wasp. This object contains user session information, as well as information about entities. The examples here won't use the context for simplicity purposes. You can read more about it in the section about using entities in APIs.
JavaScript
TypeScript
src/apis.js
export const fooBar = (req, res, context) => {
res.set("Access-Control-Allow-Origin", "*"); // Example of modifying headers to override Wasp default CORS middleware.
res.json({ msg: `Hello, ${context.user ? "registered user" : "stranger"}!` });
};
Using the API
Using the API externally
To use the API externally, you simply call the endpoint using the method and path you used.
For example, if your app is running at https://example.com then from the above you could issue a GET to https://example/com/foo/callback (in your browser, Postman, curl, another web service, etc.).
Using the API from the Client
To use the API from your client, including with auth support, you can import the Axios wrapper from wasp/client/api and invoke a call. For example:
JavaScript
TypeScript
src/pages/SomePage.jsx
import React, { useEffect } from "react";
import { api } from "wasp/client/api";
async function fetchCustomRoute() {
const res = await api.get("/foo/bar");
console.log(res.data);
}
export const Foo = () => {
useEffect(() => {
fetchCustomRoute();
}, []);
return <>// ...</>;
};
Making Sure CORS Works
APIs are designed to be as flexible as possible, hence they don't utilize the default middleware like Operations do. As a result, to use these APIs on the client side, you must ensure that CORS (Cross-Origin Resource Sharing) is enabled.
You can do this by defining custom middleware for your APIs in the Wasp file.
JavaScript
TypeScript
For example, an apiNamespace is a simple declaration used to apply some middlewareConfigFn to all APIs under some specific path:
main.wasp
apiNamespace fooBar {
middlewareConfigFn: import { fooBarNamespaceMiddlewareFn } from "@src/apis",
path: "/foo"
}
And then in the implementation file:
src/apis.js
export const apiMiddleware = (config) => {
return config;
};
We are returning the default middleware which enables CORS for all APIs under the /foo path.
For more information about middleware configuration, please see: Middleware Configuration
Using Entities in APIs
In many cases, resources used in APIs will be Entities. To use an Entity in your API, add it to the api declaration in Wasp:
JavaScript
TypeScript
main.wasp
api fooBar {
fn: import { fooBar } from "@src/apis",
entities: [Task],
httpRoute: (GET, "/foo/bar")
}
Wasp will inject the specified Entity into the APIs context argument, giving you access to the Entity's Prisma API:
JavaScript
TypeScript
src/apis.js
export const fooBar = (req, res, context) => {
res.json({ count: await context.entities.Task.count() });
};
The object context.entities.Task exposes prisma.task from Prisma's CRUD API.
API Reference
JavaScript
TypeScript
main.wasp
api fooBar {
fn: import { fooBar } from "@src/apis",
httpRoute: (GET, "/foo/bar"),
entities: [Task],
auth: true,
middlewareConfigFn: import { apiMiddleware } from "@src/apis"
}
The api declaration has the following fields:
fn: ExtImport required
The import statement of the APIs NodeJs implementation.
httpRoute: (HttpMethod, string) required
The HTTP (method, path) pair, where the method can be one of:
ALL, GET, POST, PUT or DELETE
and path is an Express path string.
entities: [Entity]
A list of entities you wish to use inside your API. You can read more about it here.
auth: bool
If auth is enabled, this will default to true and provide a context.user object. If you do not wish to attempt to parse the JWT in the Authorization Header, you should set this to false.
middlewareConfigFn: ExtImport
The import statement to an Express middleware config function for this API. See more in middleware section of the docs. | https://wasp-lang.dev/docs/advanced/apis |
|
2 | null | 2024-04-23T15:15:13.819Z | https://wasp-lang.dev/docs/0.11.8/vision | https://wasp-lang.dev/docs/vision | http | ## Vision
With Wasp, we want to make developing web apps easy and enjoyable, for novices and experts in web development alike.
Ideal we are striving for is that programming in Wasp feels like describing an app using a human language - like writing a specification document where you describe primarily your requirements and as little implementation details as possible. Creating a new production-ready web app should be easy and deploying it to production should be straightforward.
That is why we believe Wasp needs to be a programming language (DSL) and not a library - we want to capture all parts of the web app into one integrated system that is perfectly tailored just for that purpose.
On the other hand, we believe that trying to capture every single detail in one language would not be reasonable. There are solutions out there that work very well for the specific task they aim to solve (React for web components, CSS/HTML for design/markup, JS/TS for logic, ...) and we don't want to replace them with Wasp. Instead, we see Wasp as a declarative "glue" code uniting all these specific solutions and providing a higher-level notion of the web app above them.
Wasp is still early in its development and therefore far from where we imagine it will be in the future. This is what we imagine:
* **Declarative, static language** with simple basic rules and **that understands a lot of web app concepts** - "horizontal language". Supports multiple files/modules, libraries.
* **Integrates seamlessly with the most popular technologies** for building specific, more complex parts of the web app (React, CSS, JS, ...). They can be used inline (mixed with Wasp code) or provided via external files.
* **Has hatches (escape mechanisms) that allow you to customize your web app** in all the right places, but remain hidden until you need them.
* **Entity (data model) is a first-class citizen** - defined via custom Wasp syntax and it integrates very closely with the rest of the features, serving as one of the central concepts around which everything is built.
* **Out of the box** support for CRUD UI based on the Entities, to get you quickly going, but also customizable to some level.
* **"Smart" operations (queries and actions)** that in most cases automatically figure out when to update, and if not it is easy to define custom logic to compensate for that. User worries about client-server gap as little as possible.
* Support, directly in Wasp, for **declaratively defining simple components and operations**.
* Besides Wasp as a programming language, there will also be a **visual builder that generates/edits Wasp code**, allowing non-developers to participate in development. Since Wasp is declarative, we imagine such builder to naturally follow from Wasp language.
* **Server side rendering, caching, packaging, security**, ... -> all those are taken care of by Wasp. You tell Wasp what you want, and Wasp figures out how to do it.
* As **simple deployment to production/staging** as it gets.
* While it comes with the official implementation(s), **Wasp language will not be coupled with the single implementation**. Others can provide implementations that compile to different web app stacks. | null | https://wasp-lang.dev/docs/0.11.8/vision | With Wasp, we want to make developing web apps easy and enjoyable, for novices and experts in web development alike. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ececadabc07fa-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:13 GMT | Tue, 23 Apr 2024 15:25:13 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=cDeT9n2KwLHCrIo8tO8pckpZ0cQ%2BTR%2BCdF%2BCGD8hC8YBq43dedfFwqeiLi%2F4uqPpmexcVWNrAmaZKKFKY3hMFhg%2BTCe%2F5thjujDZTi1xNub1%2BsLmBeUrOpr31o7zIGP%2F"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 4be19ba071f8b4adebe7d318088298c591984cdd | null | 5336:16F6:A29E3:E0557:6627D081 | HIT | MISS | cache-iad-kiad7000044-IAD | S1713885314.752178,VS0,VE52 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/vision | og:url | Vision | Wasp | og:title | With Wasp, we want to make developing web apps easy and enjoyable, for novices and experts in web development alike. | og:description | Vision | Wasp | null | Vision
With Wasp, we want to make developing web apps easy and enjoyable, for novices and experts in web development alike.
Ideal we are striving for is that programming in Wasp feels like describing an app using a human language - like writing a specification document where you describe primarily your requirements and as little implementation details as possible. Creating a new production-ready web app should be easy and deploying it to production should be straightforward.
That is why we believe Wasp needs to be a programming language (DSL) and not a library - we want to capture all parts of the web app into one integrated system that is perfectly tailored just for that purpose.
On the other hand, we believe that trying to capture every single detail in one language would not be reasonable. There are solutions out there that work very well for the specific task they aim to solve (React for web components, CSS/HTML for design/markup, JS/TS for logic, ...) and we don't want to replace them with Wasp. Instead, we see Wasp as a declarative "glue" code uniting all these specific solutions and providing a higher-level notion of the web app above them.
Wasp is still early in its development and therefore far from where we imagine it will be in the future. This is what we imagine:
Declarative, static language with simple basic rules and that understands a lot of web app concepts - "horizontal language". Supports multiple files/modules, libraries.
Integrates seamlessly with the most popular technologies for building specific, more complex parts of the web app (React, CSS, JS, ...). They can be used inline (mixed with Wasp code) or provided via external files.
Has hatches (escape mechanisms) that allow you to customize your web app in all the right places, but remain hidden until you need them.
Entity (data model) is a first-class citizen - defined via custom Wasp syntax and it integrates very closely with the rest of the features, serving as one of the central concepts around which everything is built.
Out of the box support for CRUD UI based on the Entities, to get you quickly going, but also customizable to some level.
"Smart" operations (queries and actions) that in most cases automatically figure out when to update, and if not it is easy to define custom logic to compensate for that. User worries about client-server gap as little as possible.
Support, directly in Wasp, for declaratively defining simple components and operations.
Besides Wasp as a programming language, there will also be a visual builder that generates/edits Wasp code, allowing non-developers to participate in development. Since Wasp is declarative, we imagine such builder to naturally follow from Wasp language.
Server side rendering, caching, packaging, security, ... -> all those are taken care of by Wasp. You tell Wasp what you want, and Wasp figures out how to do it.
As simple deployment to production/staging as it gets.
While it comes with the official implementation(s), Wasp language will not be coupled with the single implementation. Others can provide implementations that compile to different web app stacks. | https://wasp-lang.dev/docs/0.11.8/vision |
|
2 | null | 2024-04-23T15:15:14.185Z | https://wasp-lang.dev/docs/0.11.8/contributing | https://wasp-lang.dev/docs/contributing | http | Version: 0.11.8
## Contributing
Any way you want to contribute is a good way, and we'd be happy to meet you! A single entry point for all contributors is the [CONTRIBUTING.md](https://github.com/wasp-lang/wasp/blob/main/CONTRIBUTING.md) file in our Github repo. All the requirements and instructions are there, so please check [CONTRIBUTING.md](https://github.com/wasp-lang/wasp/blob/main/CONTRIBUTING.md) for more details.
Some side notes to make your journey easier:
1. Join us on [Discord](https://discord.gg/rzdnErX) and let's talk! We can discuss language design, new/existing features, and weather, or you can tell us how you feel about Wasp :).
2. Wasp's compiler is built with Haskell. That means you'll need to be somewhat familiar with this language if you'd like to contribute to the compiler itself. But Haskell is just a part of Wasp, and you can contribute to lot of parts that require web dev skills, either by coding or by suggesting how to improve Wasp and its design as a web framework. If you don't have Haskell knowledge (or any dev experience at all) - no problem. There are a lot of JS-related tasks and documentation updates as well!
3. If there's something you'd like to bring to our attention, go to [docs GitHub repo](https://github.com/wasp-lang/wasp) and make an issue/PR!
Happy hacking! | null | https://wasp-lang.dev/docs/0.11.8/contributing | Any way you want to contribute is a good way, and we'd be happy to meet you! A single entry point for all contributors is the CONTRIBUTING.md file in our Github repo. All the requirements and instructions are there, so please check CONTRIBUTING.md for more details. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ececd5e4a9c4e-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:14 GMT | Tue, 23 Apr 2024 15:25:14 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=pArXCI9ytbkojULHtgNx8r5ATyktihxeOoZ77v%2BIIxSEWVqJoT7AAhKe1y6woLTpERJRF8YdUi4HcH02Ck%2FlXZHqpw9CPOkIRKcOqgqKPv9gVyfZ1Gc33yTTAM8hkzE0"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 5cde723aeb30614d58ff3f759f1ea6671b7cfa71 | null | 4696:3293B5:37E85FA:42EE1F0:6627D081 | HIT | MISS | cache-iad-kiad7000173-IAD | S1713885314.159685,VS0,VE12 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/contributing | og:url | Contributing | Wasp | og:title | Any way you want to contribute is a good way, and we'd be happy to meet you! A single entry point for all contributors is the CONTRIBUTING.md file in our Github repo. All the requirements and instructions are there, so please check CONTRIBUTING.md for more details. | og:description | Contributing | Wasp | null | Version: 0.11.8
Contributing
Any way you want to contribute is a good way, and we'd be happy to meet you! A single entry point for all contributors is the CONTRIBUTING.md file in our Github repo. All the requirements and instructions are there, so please check CONTRIBUTING.md for more details.
Some side notes to make your journey easier:
Join us on Discord and let's talk! We can discuss language design, new/existing features, and weather, or you can tell us how you feel about Wasp :).
Wasp's compiler is built with Haskell. That means you'll need to be somewhat familiar with this language if you'd like to contribute to the compiler itself. But Haskell is just a part of Wasp, and you can contribute to lot of parts that require web dev skills, either by coding or by suggesting how to improve Wasp and its design as a web framework. If you don't have Haskell knowledge (or any dev experience at all) - no problem. There are a lot of JS-related tasks and documentation updates as well!
If there's something you'd like to bring to our attention, go to docs GitHub repo and make an issue/PR!
Happy hacking! | https://wasp-lang.dev/docs/0.11.8/contributing |
|
2 | null | 2024-04-23T15:15:14.244Z | https://wasp-lang.dev/docs/0.12.0/contributing | https://wasp-lang.dev/docs/contributing | http | Version: 0.12.0
## Contributing
Any way you want to contribute is a good way, and we'd be happy to meet you! A single entry point for all contributors is the [CONTRIBUTING.md](https://github.com/wasp-lang/wasp/blob/main/CONTRIBUTING.md) file in our Github repo. All the requirements and instructions are there, so please check [CONTRIBUTING.md](https://github.com/wasp-lang/wasp/blob/main/CONTRIBUTING.md) for more details.
Some side notes to make your journey easier:
1. Join us on [Discord](https://discord.gg/rzdnErX) and let's talk! We can discuss language design, new/existing features, and weather, or you can tell us how you feel about Wasp :).
2. Wasp's compiler is built with Haskell. That means you'll need to be somewhat familiar with this language if you'd like to contribute to the compiler itself. But Haskell is just a part of Wasp, and you can contribute to lot of parts that require web dev skills, either by coding or by suggesting how to improve Wasp and its design as a web framework. If you don't have Haskell knowledge (or any dev experience at all) - no problem. There are a lot of JS-related tasks and documentation updates as well!
3. If there's something you'd like to bring to our attention, go to [docs GitHub repo](https://github.com/wasp-lang/wasp) and make an issue/PR!
Happy hacking! | null | https://wasp-lang.dev/docs/0.12.0/contributing | Any way you want to contribute is a good way, and we'd be happy to meet you! A single entry point for all contributors is the CONTRIBUTING.md file in our Github repo. All the requirements and instructions are there, so please check CONTRIBUTING.md for more details. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ececdc9666ff5-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:14 GMT | Tue, 23 Apr 2024 15:25:14 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=9iYuW5HLB4X1KkpgBq2W43Ilf8Hm7ahvG7BXu%2BQKWEoLHm1hRCgyMNAP7P0ql29MY5hS5YlLFw%2BfaQ%2F8lG98znUxqNc68FcKnYUlyAS%2BNbmhbsPSVlAo2PagsT0KkuUB"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 079697422dd0d4e0bfe3aba48354646e2d55deac | null | 9040:1A89F:3BDADCD:46E0E0F:6627D082 | HIT | MISS | cache-iad-kiad7000052-IAD | S1713885314.224708,VS0,VE9 | null | null | en | og:image | https://wasp-lang.dev/docs/0.12.0/contributing | og:url | Contributing | Wasp | og:title | Any way you want to contribute is a good way, and we'd be happy to meet you! A single entry point for all contributors is the CONTRIBUTING.md file in our Github repo. All the requirements and instructions are there, so please check CONTRIBUTING.md for more details. | og:description | Contributing | Wasp | null | Version: 0.12.0
Contributing
Any way you want to contribute is a good way, and we'd be happy to meet you! A single entry point for all contributors is the CONTRIBUTING.md file in our Github repo. All the requirements and instructions are there, so please check CONTRIBUTING.md for more details.
Some side notes to make your journey easier:
Join us on Discord and let's talk! We can discuss language design, new/existing features, and weather, or you can tell us how you feel about Wasp :).
Wasp's compiler is built with Haskell. That means you'll need to be somewhat familiar with this language if you'd like to contribute to the compiler itself. But Haskell is just a part of Wasp, and you can contribute to lot of parts that require web dev skills, either by coding or by suggesting how to improve Wasp and its design as a web framework. If you don't have Haskell knowledge (or any dev experience at all) - no problem. There are a lot of JS-related tasks and documentation updates as well!
If there's something you'd like to bring to our attention, go to docs GitHub repo and make an issue/PR!
Happy hacking! | https://wasp-lang.dev/docs/0.12.0/contributing |
|
2 | null | 2024-04-23T15:15:14.262Z | https://wasp-lang.dev/docs/0.12.0/telemetry | https://wasp-lang.dev/docs/telemetry | http | ```
{ // Randomly generated, non-identifiable UUID representing a user. "distinct_id": "bf3fa7a8-1c11-4f82-9542-ec1a2d28786b", // Non-identifiable hash representing a project. "project_hash": "6d7e561d62b955d1", // True if command was `wasp build`, false otherwise. "is_build": true, // Captures `wasp deploy ...` args, but only those from the limited, pre-defined list of keywords. // Those are "fly", "setup", "create-db", "deploy" and "cmd". Everything else is ommited. "deploy_cmd_args": "fly;deploy", "wasp_version": "0.1.9.1", "os": "linux", // "CI" if running on CI, and whatever is the content of "WASP_TELEMETRY_CONTEXT" env var. // We use this to track when execution is happening in some special context, like on Gitpod, Replit or similar. "context": "CI"}
``` | null | https://wasp-lang.dev/docs/0.12.0/telemetry | Overview | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ececd8bae8f23-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:14 GMT | Tue, 23 Apr 2024 15:25:14 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=Ypb8YahapuWBDt3gMvw7Lum%2BUfFvJ8kQHWLrSHq0%2BFeNxj5%2FCMk9KFQUYoyntJIeKs54UAsNdWW%2BN8pD6tHqanVtk5gqwRtIS0P%2BNIwgiCtlh1YBpqtPF7%2F2o6KWSqqw"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | b1b7e2c45b262a855fe472e58465f288908f8b6d | null | 6CD0:209F3A:3A80CB1:45876B1:6627D082 | HIT | MISS | cache-iad-kiad7000171-IAD | S1713885314.203278,VS0,VE47 | null | null | en | og:image | https://wasp-lang.dev/docs/0.12.0/telemetry | og:url | Telemetry | Wasp | og:title | Overview | og:description | Telemetry | Wasp | null | {
// Randomly generated, non-identifiable UUID representing a user.
"distinct_id": "bf3fa7a8-1c11-4f82-9542-ec1a2d28786b",
// Non-identifiable hash representing a project.
"project_hash": "6d7e561d62b955d1",
// True if command was `wasp build`, false otherwise.
"is_build": true,
// Captures `wasp deploy ...` args, but only those from the limited, pre-defined list of keywords.
// Those are "fly", "setup", "create-db", "deploy" and "cmd". Everything else is ommited.
"deploy_cmd_args": "fly;deploy",
"wasp_version": "0.1.9.1",
"os": "linux",
// "CI" if running on CI, and whatever is the content of "WASP_TELEMETRY_CONTEXT" env var.
// We use this to track when execution is happening in some special context, like on Gitpod, Replit or similar.
"context": "CI"
} | https://wasp-lang.dev/docs/0.12.0/telemetry |
|
2 | null | 2024-04-23T15:15:15.549Z | https://wasp-lang.dev/docs/0.11.8/telemetry | https://wasp-lang.dev/docs/telemetry | http | ```
{ // Randomly generated, non-identifiable UUID representing a user. "distinct_id": "bf3fa7a8-1c11-4f82-9542-ec1a2d28786b", // Non-identifiable hash representing a project. "project_hash": "6d7e561d62b955d1", // True if command was `wasp build`, false otherwise. "is_build": true, // Captures `wasp deploy ...` args, but only those from the limited, pre-defined list of keywords. // Those are "fly", "setup", "create-db", "deploy" and "cmd". Everything else is ommited. "deploy_cmd_args": "fly;deploy", "wasp_version": "0.1.9.1", "os": "linux", // "CI" if running on CI, and whatever is the content of "WASP_TELEMETRY_CONTEXT" env var. // We use this to track when execution is happening in some special context, like on Gitpod, Replit or similar. "context": "CI"}
``` | null | https://wasp-lang.dev/docs/0.11.8/telemetry | Overview | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878eced5bb580828-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:15 GMT | Tue, 23 Apr 2024 15:25:15 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=UW0U6Z%2FJ9hWXDBiRjtuomygPnT%2FPAf2wL%2FxaNytQnNM5TlkF6vlrna5GHgKwrTXR6AJHrPRmbzAWq%2Bj%2B2RJqA2KvbJGE18BDEz3TSbmiPtvOj0AySvyXha55DSYxQibG"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 22906800db5899b09c75661bb21aeb3a8a8a1ee0 | null | B1C0:209F3A:3A80DEF:458781E:6627D082 | HIT | MISS | cache-iad-kiad7000152-IAD | S1713885316.521132,VS0,VE11 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/telemetry | og:url | Telemetry | Wasp | og:title | Overview | og:description | Telemetry | Wasp | null | {
// Randomly generated, non-identifiable UUID representing a user.
"distinct_id": "bf3fa7a8-1c11-4f82-9542-ec1a2d28786b",
// Non-identifiable hash representing a project.
"project_hash": "6d7e561d62b955d1",
// True if command was `wasp build`, false otherwise.
"is_build": true,
// Captures `wasp deploy ...` args, but only those from the limited, pre-defined list of keywords.
// Those are "fly", "setup", "create-db", "deploy" and "cmd". Everything else is ommited.
"deploy_cmd_args": "fly;deploy",
"wasp_version": "0.1.9.1",
"os": "linux",
// "CI" if running on CI, and whatever is the content of "WASP_TELEMETRY_CONTEXT" env var.
// We use this to track when execution is happening in some special context, like on Gitpod, Replit or similar.
"context": "CI"
} | https://wasp-lang.dev/docs/0.11.8/telemetry |
|
2 | null | 2024-04-23T15:15:16.316Z | https://wasp-lang.dev/docs/0.11.8/contact | https://wasp-lang.dev/docs/contact | http | Version: 0.11.8
## Contact
You can find us on [Discord](https://discord.gg/rzdnErX) or you can reach out to us via email at [\[email protected\]](https://wasp-lang.dev/cdn-cgi/l/email-protection#593130192e382a29743538373e773d3c2f). | null | https://wasp-lang.dev/docs/0.11.8/contact | You can find us on Discord or you can reach out to us via email at hi@wasp-lang.dev. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878eceda8b843985-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:16 GMT | Tue, 23 Apr 2024 15:25:16 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=shlwWqBOEWHLk0Le5HsOiD%2BQsSIsQLyM4Y3nI6FOwO5mEfwpTQF%2FgeOByzguxxJk3AoV8jEtlqzxPa%2B0Tl84UwRz7uc5xVBdUeNMOPmFb%2F3Y%2F2FE8wh437aTYQjC7Sj7"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 64276874474203ad315e2b7dd9f34ce4c974181b | null | 88BC:180046:3C2F517:4735B4D:6627D084 | null | MISS | cache-iad-kiad7000171-IAD | S1713885316.264475,VS0,VE38 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/contact | og:url | Contact | Wasp | og:title | You can find us on Discord or you can reach out to us via email at hi@wasp-lang.dev. | og:description | Contact | Wasp | null | Version: 0.11.8
Contact
You can find us on Discord or you can reach out to us via email at [email protected]. | https://wasp-lang.dev/docs/0.11.8/contact |
|
2 | null | 2024-04-23T15:15:16.230Z | https://wasp-lang.dev/docs/0.12.0/contact | https://wasp-lang.dev/docs/contact | http | Version: 0.12.0
## Contact
You can find us on [Discord](https://discord.gg/rzdnErX) or you can reach out to us via email at [\[email protected\]](https://wasp-lang.dev/cdn-cgi/l/email-protection#c0a8a980b7a1b3b0edaca1aea7eea4a5b6). | null | https://wasp-lang.dev/docs/0.12.0/contact | You can find us on Discord or you can reach out to us via email at hi@wasp-lang.dev. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878eceda0b285944-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:16 GMT | Tue, 23 Apr 2024 15:25:16 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=HE4otgMd5jG5xTism1g1aKyK2TO5pDuC7rVYqXwTCJl5wAUgRntPG3h9n2amDwntbJAPNNhPImdwcjv1%2B2x%2FWz5yNy6ifBX%2FWlTJ%2BRMQWGFOxnIHBQxm%2FkUi3v4d7EA5"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | fa011f99b108b534ff90dabf7fc2093ff020ed15 | null | 6170:52242:3974B30:447B106:6627D083 | HIT | MISS | cache-iad-kiad7000158-IAD | S1713885316.200739,VS0,VE9 | null | null | en | og:image | https://wasp-lang.dev/docs/0.12.0/contact | og:url | Contact | Wasp | og:title | You can find us on Discord or you can reach out to us via email at hi@wasp-lang.dev. | og:description | Contact | Wasp | null | Version: 0.12.0
Contact
You can find us on Discord or you can reach out to us via email at [email protected]. | https://wasp-lang.dev/docs/0.12.0/contact |
|
2 | null | 2024-04-23T15:15:16.486Z | https://wasp-lang.dev/docs/0.12.0/migrate-from-0-11-to-0-12 | https://wasp-lang.dev/docs/migrate-from-0-11-to-0-12 | http | ## What's new in Wasp 0.12.0?[](#whats-new-in-wasp-0120 "Direct link to What's new in Wasp 0.12.0?")
### New project structure[](#new-project-structure "Direct link to New project structure")
Here's a file tree of a fresh Wasp project created with the previous version of Wasp. More precisely, this is what you'll get if you run `wasp new myProject` using Wasp 0.11.x:
```
.├── .gitignore├── main.wasp├── src│ ├── client│ │ ├── Main.css│ │ ├── MainPage.jsx│ │ ├── react-app-env.d.ts│ │ ├── tsconfig.json│ │ └── waspLogo.png│ ├── server│ │ └── tsconfig.json│ ├── shared│ │ └── tsconfig.json│ └── .waspignore└── .wasproot
```
Compare that with the file tree of a fresh Wasp project created with Wasp 0.12.0. In other words, this is what you will get by running `wasp new myProject` from this point onwards:
```
.├── .gitignore├── main.wasp├── package.json├── public│ └── .gitkeep├── src│ ├── Main.css│ ├── MainPage.jsx│ ├── queries.ts│ ├── vite-env.d.ts│ ├── .waspignore│ └── waspLogo.png├── tsconfig.json├── vite.config.ts└── .wasproot
```
The main differences are:
* The server/client code separation is no longer necessary. You can now organize your code however you want, as long as it's inside the `src` directory.
* All external imports in your Wasp file must have paths starting with `@src` (e.g., `import foo from '@src/bar.js'`) where `@src` refers to the `src` directory in your project root. The paths can no longer start with `@server` or `@client`.
* Your project now features a top-level `public` dir. Wasp will publicly serve all the files it finds in this directory. Read more about it [here](https://wasp-lang.dev/docs/project/static-assets).
Our [Overview docs](https://wasp-lang.dev/docs/0.12.0/tutorial/project-structure) explain the new structure in detail, while this page provides a [quick guide](#migrating-your-project-to-the-new-structure) for migrating existing projects.
### New auth[](#new-auth "Direct link to New auth")
In Wasp 0.11.X, authentication was based on the `User` model which the developer needed to set up properly and take care of the auth fields like `email` or `password`.
main.wasp
```
app myApp { wasp: { version: "^0.11.0" }, title: "My App", auth: { userEntity: User, externalAuthEntity: SocialLogin, methods: { gitHub: {} }, onAuthFailedRedirectTo: "/login" },}entity User {=psl id Int @id @default(autoincrement()) username String @unique password String externalAuthAssociations SocialLogin[]psl=}entity SocialLogin {=psl id Int @id @default(autoincrement()) provider String providerId String user User @relation(fields: [userId], references: [id], onDelete: Cascade) userId Int createdAt DateTime @default(now()) @@unique([provider, providerId, userId])psl=}
```
From 0.12.X onwards, authentication is based on the auth models which are automatically set up by Wasp. You don't need to take care of the auth fields anymore.
The `User` model is now just a business logic model and you use it for storing the data that is relevant for your app.
main.wasp
```
app myApp { wasp: { version: "^0.12.0" }, title: "My App", auth: { userEntity: User, methods: { gitHub: {} }, onAuthFailedRedirectTo: "/login" },}entity User {=psl id Int @id @default(autoincrement())psl=}
```
Regression Note: Multiple Auth Identities per User
With our old auth implementation, if you were using both Google and email auth methods, your users could sign up with Google first and then, later on, reset their password and therefore also enable logging in with their email and password. This was the only way in which a single user could have multiple login methods at the same time (Google and email).
This is not possible anymore. **The new auth system doesn't support multiple login methods per user at the moment**. We do plan to add this soon though, with the introduction of the [account merging feature](https://github.com/wasp-lang/wasp/issues/954).
If you have any users that have both Google and email login credentials at the same time, you will have to pick only one of those for that user to keep when migrating them.
Regression Note: `_waspCustomValidations` is deprecated
Auth field customization is no longer possible using the `_waspCustomValidations` on the `User` entity. This is a part of auth refactoring that we are doing to make it easier to customize auth. We will be adding more customization options in the future.
You can read more about the new auth system in the [Auth Entities](https://wasp-lang.dev/docs/0.12.0/auth/entities) section.
## How to Migrate?[](#how-to-migrate "Direct link to How to Migrate?")
These instructions are for migrating your app from Wasp `0.11.X` to Wasp `0.12.X`, meaning they will work for all minor releases that fit this pattern (e.g., the guide applies to `0.12.0`, `0.12.1`, ...).
The guide consists of two big steps:
1. Migrating your Wasp project to the new structure.
2. Migrating to the new auth.
If you get stuck at any point, don't hesitate to ask for help on [our Discord server](https://discord.gg/rzdnErX).
### Migrating Your Project to the New Structure[](#migrating-your-project-to-the-new-structure "Direct link to Migrating Your Project to the New Structure")
You can easily migrate your old Wasp project to the new structure by following a series of steps. Assuming you have a project called `foo` inside the directory `foo`, you should:
0. **Install the latest `0.12.x` version** of Wasp.
```
curl -sSL https://get.wasp-lang.dev/installer.sh | sh -s
```
1. Make sure to **backup or save your project** before starting the procedure (e.g., by committing it to source control or creating a copy).
2. **Position yourself in the terminal** in the directory that is a parent of your wasp project directory (so one level above: if you do `ls`, you should see your wasp project dir listed).
3. **Run the migration script** (replace `foo` at the end with the name of your Wasp project directory) and follow the instructions:
In case the migration script doesn't work well for you, you can do the same steps manually, as described here:
1. Rename your project's root directory to something like `foo_old`.
2. Create a new project by running `wasp new foo`.
3. Delete all files of `foo/src` except `vite-env.d.ts`.
4. If `foo_old/src/client/public` exists and contains any files, copy those files into `foo/public`.
5. Copy the contents of `foo_old/src` into `foo/src`. `foo/src` should now contain `vite-env.d.ts`, `.waspignore`, and three subdirectories (`server`, `client`, and `shared`). Don't change anything about this structure yet.
6. Delete redundant files and folders from `foo/src`:
* `foo/src/.waspignore` - A new version of this file already exists at the top level.
* `foo/src/client/vite-env.d.ts` - A new version of this file already exists at the top level.
* `foo/src/client/tsconfig.json` - A new version of this file already exists at the top level.
* `foo/src/server/tsconfig.json` - A new version of this file already exists at the top level.
* `foo/src/shared/tsconfig.json` - A new version of this file already exists at the top level.
* `foo/src/client/public` - You've moved all the files from this directory in step 5.
7. Update all the `@wasp` imports in your JS(X)/TS(X) source files in the `src/` dir.
For this, we prepared a special script that will rewrite these imports automatically for you.
Before doing this step, as the script will modify your JS(X)/TS(X) files in place, we advise committing all changes you have so far, so you can then both easily inspect the import rewrites that our script did (with `git diff`) and also revert them if something went wrong.
To run the import-rewriting script, make sure you are in the root dir of your wasp project, and then run
```
npx [email protected] -t https://raw.githubusercontent.com/wasp-lang/wasp-codemod/main/src/transforms/imports-from-0-11-to-0-12.ts --extensions=js,ts,jsx,tsx src/
```
Then, check the changes it did, in case some kind of manual intervention is needed (in which case you should see TODO comments generated by the script).
Alternatively, you can find all the mappings of old imports to the new ones in [this table](https://docs.google.com/spreadsheets/d/1QW-_16KRGTOaKXx9NYUtjk6m2TQ0nUMOA74hBthTH3g/edit#gid=1725669920) and use it to fix some/all of them manually.
8. Replace the Wasp file in `foo` (i.e., `main.wasp`) with the Wasp file from `foo_old`
9. Change the Wasp version field in your Wasp file (now residing in `foo`) to `"^0.12.0"`.
10. Correct external imports in your Wasp file (now residing in `foo`). imports. You can do this by running search-and-replace inside the file:
* Change all occurrences of `@server` to `@src/server`
* Change all occurrences of `@client` to `@src/client`
For example, if you previously had something like:
```
page LoginPage { // This previously resolved to src/client/LoginPage.js component: import Login from "@client/LoginPage"}// ...query getTasks { // This previously resolved to src/server/queries.js fn: import { getTasks } from "@server/queries.js",}
```
You should change it to:
```
page LoginPage { // This now resolves to src/client/LoginPage.js component: import Login from "@src/client/LoginPage"}// ...query getTasks { // This now resolves to src/server/queries.js fn: import { getTasks } from "@src/server/queries.js",}
```
Do this for all external imports in your `.wasp` file. After you're done, there shouldn't be any occurrences of strings `"@server"` or `"@client"`
11. Take all the dependencies from `app.dependencies` declaration in `foo/main.wasp` and move them to `foo/package.json`. Make sure to remove the `app.dependencies` field from `foo/main.wasp`.
For example, if `foo_old/main.wasp` had:
```
app Foo { // ... dependencies: [ ('redux', '^4.0.5'), ('reacjt-redux', '^7.1.3')];}
```
Your `package.json` in `foo` should now list these dependencies (Wasp already generated most of the file, you just have to list additional dependencies).
```
{ "name": "foo", "dependencies": { "wasp": "file:.wasp/out/sdk/wasp", "react": "^18.2.0", "redux": "^4.0.5", "reactjs-redux": "^7.1.3" }, "devDependencies": { "typescript": "^5.1.0", "vite": "^4.3.9", "@types/react": "^18.0.37", "prisma": "4.16.2" }}
```
12. Copy all lines you might have added to `foo_old/.gitignore` into `foo/.gitignore`
13. Copy the rest of the top-level files and folders (all of them except for `.gitignore`, `main.wasp` and `src/`) in `foo_old/` into `foo/` (overwrite the existing files in `foo`).
14. Run `wasp clean` in `foo`.
15. Delete the `foo_old` directory.
That's it! You now have a properly structured Wasp 0.12.0 project in the `foo` directory. Your app probably doesn't quite work yet due to some other changes in Wasp 0.12.0, but we'll get to that in the next sections.
### Migrating declaration names[](#migrating-declaration-names "Direct link to Migrating declaration names")
Wasp 0.12.0 adds a casing constraints when naming Queries, Actions, Jobs, and Entities in the `main.wasp` file.
The following casing conventions have now become mandatory:
* Operation (i.e., Query and Action) names must begin with a lowercase letter: `query getTasks {...}`, `action createTask {...}`.
* Job names must begin with a lowercase letter: `job sendReport {...}`.
* Entity names must start with an uppercase letter: `entity Task {...}`.
### Migrating the Tailwind Setup[](#migrating-the-tailwind-setup "Direct link to Migrating the Tailwind Setup")
note
If you don't use Tailwind in your project, you can skip this section.
There is a small change in how the `tailwind.config.cjs` needs to be defined in Wasp 0.12.0.
You'll need to wrap all your paths in the `content` field with the `resolveProjectPath` function. This makes sure that the paths are resolved correctly when generating your CSS.
Here's how you can do it:
* Before
* After
tailwind.config.cjs
```
/** @type {import('tailwindcss').Config} */module.exports = { content: [ './src/**/*.{js,jsx,ts,tsx}', ], theme: { extend: {}, }, plugins: [],}
```
### Default Server Dockerfile Changed[](#default-server-dockerfile-changed "Direct link to Default Server Dockerfile Changed")
note
If you didn't customize your Dockerfile or had a custom build process for the Wasp server, you can skip this section.
Between Wasp 0.11.X and 0.12.X, the Dockerfile that Wasp generates for you for deploying the server has changed. If you defined a custom Dockerfile in your project root dir or in any other way relied on its contents, you'll need to update it to incorporate the changes that Wasp 0.12.X made.
We suggest that you temporarily move your custom Dockerfile to a different location, then run `wasp start` to generate the new Dockerfile. Check out the `.wasp/out/Dockerfile` to see the new Dockerfile and what changes you need to make. You'll probably need to copy some of the changes from the new Dockerfile to your custom one to make your app work with Wasp 0.12.X.
### Migrating to the New Auth[](#migrating-to-the-new-auth "Direct link to Migrating to the New Auth")
As shown in [the previous section](#new-auth), Wasp significantly changed how authentication works in version 0.12.0. This section leads you through migrating your app from Wasp 0.11.X to Wasp 0.12.X.
Migrating your existing app to the new auth system is a two-step process:
1. Migrate to the new auth system
2. Clean up the old auth system
Migrating a deployed app
While going through these steps, we will focus first on doing the changes locally (including your local development database).
Once we confirm everything works well locally, we will apply the same changes to the deployed app (including your production database).
**We'll put extra info for migrating a deployed app in a box like this one.**
#### 1\. Migrate to the New Auth System[](#1-migrate-to-the-new-auth-system "Direct link to 1. Migrate to the New Auth System")
You can follow these steps to migrate to the new auth system (assuming you already migrated the project structure to 0.12, as described [above](#migrating-your-project-to-the-new-structure)):
1. **Migrate `getUserFields` and/or `additionalSignupFields` in the `main.wasp` file to the new `userSignupFields` field.**
If you are not using them, you can skip this step.
In Wasp 0.11.X, you could define a `getUserFieldsFn` to specify extra fields that would get saved to the `User` when using Google or GitHub to sign up.
You could also define `additionalSignupFields` to specify extra fields for the Email or Username & Password signup.
In 0.12.X, we unified these two concepts into the `userSignupFields` field.
Migration for [Email](https://wasp-lang.dev/docs/auth/email) and [Username & Password](https://wasp-lang.dev/docs/auth/username-and-pass)
First, move the value of `auth.signup.additionalFields` to `auth.methods.{method}.userSignupFields` in the `main.wasp` file.
`{method}` depends on the auth method you are using. For example, if you are using the email auth method, you should move the `auth.signup.additionalFields` to `auth.methods.email.userSignupFields`.
To finish, update the JS/TS implementation to use the `defineUserSignupFields` from `wasp/server/auth` instead of `defineAdditionalSignupFields` from `@wasp/auth/index.js`.
* Before
* After
main.wasp
```
app crudTesting { // ... auth: { userEntity: User, methods: { email: {}, }, onAuthFailedRedirectTo: "/login", signup: { additionalFields: import { fields } from "@server/auth/signup.js", }, },}
```
src/server/auth/signup.ts
```
import { defineAdditionalSignupFields } from '@wasp/auth/index.js'export const fields = defineAdditionalSignupFields({ address: async (data) => { const address = data.address if (typeof address !== 'string') { throw new Error('Address is required') } if (address.length < 5) { throw new Error('Address must be at least 5 characters long') } return address },})
```
Migration for [Github](https://wasp-lang.dev/docs/auth/social-auth/github) and [Google](https://wasp-lang.dev/docs/auth/social-auth/google)
First, move the value of `auth.methods.{method}.getUserFieldsFn` to `auth.methods.{method}.userSignupFields` in the `main.wasp` file.
`{method}` depends on the auth method you are using. For example, if you are using Google auth, you should move the `auth.methods.google.getUserFieldsFn` to `auth.methods.google.userSignupFields`.
To finish, update the JS/TS implementation to use the `defineUserSignupFields` from `wasp/server/auth` and modify the code to return the fields in the format that `defineUserSignupFields` expects.
* Before
* After
main.wasp
```
app crudTesting { // ... auth: { userEntity: User, methods: { google: { getUserFieldsFn: import { getUserFields } from "@server/auth/google.js" }, }, onAuthFailedRedirectTo: "/login", },}
```
src/server/auth/google.ts
```
import type { GetUserFieldsFn } from '@wasp/types'export const getUserFields: GetUserFieldsFn = async (_context, args) => { const displayName = args.profile.displayName return { displayName }}
```
2. **Remove the `auth.methods.email.allowUnverifiedLogin` field** from your `main.wasp` file.
In Wasp 0.12.X we removed the `auth.methods.email.allowUnverifiedLogin` field to make our Email auth implementation easier to reason about. If you were using it, you should remove it from your `main.wasp` file.
3. Ensure your **local development database is running**.
4. **Do the schema migration** (create the new auth tables in the database) by running:
You should see the new `Auth`, `AuthIdentity` and `Session` tables in your database. You can use the `wasp db studio` command to open the database in a GUI and verify the tables are there. At the moment, they will be empty.
5. **Do the data migration** (move existing users from the old auth system to the new one by filling the new auth tables in the database with their data):
1. **Implement your data migration function(s)** in e.g. `src/migrateToNewAuth.ts`.
Below we prepared [examples of migration functions](#example-data-migration-functions) for each of the auth methods, for you to use as a starting point. They should be fine to use as-is, meaning you can just copy them and they are likely to work out of the box for typical use cases, but you can also modify them for your needs.
We recommend you create one function per each auth method that you use in your app.
2. **Define custom API endpoints for each migration function** you implemented.
With each data migration function below, we provided a relevant `api` declaration that you should add to your `main.wasp` file.
3. **Run the data migration function(s)** on the local development database by calling the API endpoints you defined in the previous step.
You can call the endpoint by visiting the URL in your browser, or by using a tool like `curl` or Postman.
For example, if you defined the API endpoint at `/migrate-username-and-password`, you can call it by visiting `http://localhost:3001/migrate-username-and-password` in your browser.
This should be it, you can now run `wasp db studio` again and verify that there is now relevant data in the new auth tables (`Auth` and `AuthIdentity`; `Session` should still be empty for now).
6. **Verify that the basic auth functionality works** by running `wasp start` and successfully signing up / logging in with each of the auth methods.
7. **Update your JS/TS code** to work correctly with the new auth.
You might want to use the new auth helper functions to get the `email` or `username` from a user object. For example, `user.username` might not work anymore for you, since the `username` obtained by the Username & Password auth method isn't stored on the `User` entity anymore (unless you are explicitly storing something into `user.username`, e.g. via `userSignupFields` for a social auth method like Github). Same goes for `email` from Email auth method.
Instead, you can now use `getUsername(user)` to get the username obtained from Username & Password auth method, or `getEmail(user)` to get the email obtained from Email auth method.
Read more about the helpers in the [Auth Entities - Accessing the Auth Fields](https://wasp-lang.dev/docs/0.12.0/auth/entities#accessing-the-auth-fields) section.
8. Finally, **check that your app now fully works as it worked before**. If all the above steps were done correctly, everything should be working now.
Migrating a deployed app
After successfully performing migration locally so far, and verifying that your app works as expected, it is time to also migrate our deployed app.
Before migrating your production (deployed) app, we advise you to back up your production database in case something goes wrong. Also, besides testing it in development, it's good to test the migration in a staging environment if you have one.
We will perform the production migration in 2 steps:
* Deploying the new code to production (client and server).
* Migrating the production database data.
* * *
Between these two steps, so after successfully deploying the new code to production and before migrating the production database data, your app will not be working completely: new users will be able to sign up, but existing users won't be able to log in, and already logged in users will be logged out. Once you do the second step, migrating the production database data, it will all be back to normal. You will likely want to keep the time between the two steps as short as you can.
* * *
* **First step: deploy the new code** (client and server), either via `wasp deploy` (i.e. `wasp deploy fly deploy`) or manually.
Check our [Deployment docs](https://wasp-lang.dev/docs/0.12.0/advanced/deployment/overview) for more details.
* **Second step: run the data migration functions** on the production database.
You can do this by calling the API endpoints you defined in the previous step, just like you did locally. You can call the endpoint by visiting the URL in your browser, or by using a tool like `curl` or Postman.
For example, if you defined the API endpoint at `/migrate-username-and-password`, you can call it by visiting `https://your-server-url.com/migrate-username-and-password` in your browser.
Your deployed app should be working normally now, with the new auth system.
#### 2\. Cleanup the Old Auth System[](#2-cleanup-the-old-auth-system "Direct link to 2. Cleanup the Old Auth System")
Your app should be working correctly and using new auth, but to finish the migration, we need to clean up the old auth system:
1. In `main.wasp` file, **delete auth-related fields from the `User` entity**, since with 0.12 they got moved to the internal Wasp entity `AuthIdentity`.
* This means any fields that were required by Wasp for authentication, like `email`, `password`, `isEmailVerified`, `emailVerificationSentAt`, `passwordResetSentAt`, `username`, etc.
* There are situations in which you might want to keep some of them, e.g. `email` and/or `username`, if they are still relevant for you due to your custom logic (e.g. you are populating them with `userSignupFields` upon social signup in order to have this info easily available on the `User` entity). Note that they won't be used by Wasp Auth anymore, they are here just for your business logic.
2. In `main.wasp` file, **remove the `externalAuthEntity` field from the `app.auth`** and also **remove the whole `SocialLogin` entity** if you used Google or GitHub auth.
3. **Delete the data migration function(s)** you implemented earlier (e.g. in `src/migrateToNewAuth.ts`) and also the corresponding API endpoints from the `main.wasp` file.
4. **Run `wasp db migrate-dev`** again to apply these changes and remove the redundant fields from the database.
Migrating a deployed app
After doing the steps above successfully locally and making sure everything is working, it is time to push these changes to the deployed app again.
_Deploy the app again_, either via `wasp deploy` or manually. Check our [Deployment docs](https://wasp-lang.dev/docs/0.12.0/advanced/deployment/overview) for more details.
The database migrations will automatically run on successful deployment of the server and delete the now redundant auth-related `User` columns from the database.
Your app is now fully migrated to the new auth system.
### Next Steps[](#next-steps "Direct link to Next Steps")
If you made it this far, you've completed all the necessary steps to get your Wasp app working with Wasp 0.12.x. Nice work!
Finally, since Wasp no longer requires you to separate your client source files (previously in `src/client`) from server source files (previously in `src/server`), you are now free to reorganize your project however you think is best, as long as you keep all the source files in the `src/` directory.
This section is optional, but if you didn't like the server/client separation, now's the perfect time to change it.
For example, if your `src` dir looked like this:
```
src│├── client│ ├── Dashboard.tsx│ ├── Login.tsx│ ├── MainPage.tsx│ ├── Register.tsx│ ├── Task.css│ ├── TaskLisk.tsx│ ├── Task.tsx│ └── User.tsx├── server│ ├── taskActions.ts│ ├── taskQueries.ts│ ├── userActions.ts│ └── userQueries.ts└── shared └── utils.ts
```
you can now change it to a feature-based structure (which we recommend for any project that is not very small):
```
src│├── task│ ├── actions.ts -- former taskActions.ts│ ├── queries.ts -- former taskQueries.ts│ ├── Task.css│ ├── TaskLisk.tsx│ └── Task.tsx├── user│ ├── actions.ts -- former userActions.ts│ ├── Dashboard.tsx│ ├── Login.tsx│ ├── queries.ts -- former userQueries.ts│ ├── Register.tsx│ └── User.tsx├── MainPage.tsx└── utils.ts
```
## Appendix[](#appendix "Direct link to Appendix")
### Example Data Migration Functions[](#example-data-migration-functions "Direct link to Example Data Migration Functions")
The migration functions provided below are written with the typical use cases in mind and you can use them as-is. If your setup requires additional logic, you can use them as a good starting point and modify them to your needs.
Note that all of the functions below are written to be idempotent, meaning that running a function multiple times can't hurt. This allows executing a function again in case only a part of the previous execution succeeded and also means that accidentally running it one time too much won't have any negative effects. **We recommend you keep your data migration functions idempotent**.
#### Username & Password[](#username--password "Direct link to Username & Password")
To successfully migrate the users using the Username & Password auth method, you will need to do two things:
1. Migrate the user data
Username & Password data migration function
main.wasp
```
api migrateUsernameAndPassword { httpRoute: (GET, "/migrate-username-and-password"), fn: import { migrateUsernameAndPasswordHandler } from "@src/migrateToNewAuth", entities: []}
```
src/migrateToNewAuth.ts
```
import { prisma } from "wasp/server";import { type ProviderName, type UsernameProviderData } from "wasp/server/auth";import { MigrateUsernameAndPassword } from "wasp/server/api";export const migrateUsernameAndPasswordHandler: MigrateUsernameAndPassword = async (_req, res) => { const result = await migrateUsernameAuth(); res.status(200).json({ message: "Migrated users to the new auth", result }); };async function migrateUsernameAuth(): Promise<{ numUsersAlreadyMigrated: number; numUsersNotUsingThisAuthMethod: number; numUsersMigratedSuccessfully: number;}> { const users = await prisma.user.findMany({ include: { auth: true, }, }); const result = { numUsersAlreadyMigrated: 0, numUsersNotUsingThisAuthMethod: 0, numUsersMigratedSuccessfully: 0, }; for (const user of users) { if (user.auth) { result.numUsersAlreadyMigrated++; console.log("Skipping user (already migrated) with id:", user.id); continue; } if (!user.username || !user.password) { result.numUsersNotUsingThisAuthMethod++; console.log("Skipping user (not using username auth) with id:", user.id); continue; } const providerData: UsernameProviderData = { hashedPassword: user.password, }; const providerName: ProviderName = "username"; await prisma.auth.create({ data: { identities: { create: { providerName, providerUserId: user.username.toLowerCase(), providerData: JSON.stringify(providerData), }, }, user: { connect: { id: user.id, }, }, }, }); result.numUsersMigratedSuccessfully++; } return result;}
```
2. Provide a way for users to migrate their password
There is a **breaking change between the old and the new auth in the way the password is hashed**. This means that users will need to migrate their password after the migration, as the old password will no longer work.
Since the only way users using username and password as a login method can verify their identity is by providing both their username and password (there is no email or any other info, unless you asked for it and stored it explicitly), we need to provide them a way to **exchange their old password for a new password**. One way to handle this is to inform them about the need to migrate their password (on the login page) and provide a custom page to migrate the password.
Steps to create a custom page for migrating the password
1. You will need to install the `secure-password` and `sodium-native` packages to use the old hashing algorithm:
Make sure to save the exact versions of the packages.
2. Then you'll need to create a new page in your app where users can migrate their password. You can use the following code as a starting point:
* JavaScript
* TypeScript
main.wasp
```
route MigratePasswordRoute { path: "/migrate-password", to: MigratePassword }page MigratePassword { component: import { MigratePasswordPage } from "@src/pages/MigratePassword"}
```
src/pages/MigratePassword.jsx
```
import { FormItemGroup, FormLabel, FormInput, FormError,} from "wasp/client/auth";import { useForm } from "react-hook-form";import { migratePassword } from "wasp/client/operations";import { useState } from "react";export function MigratePasswordPage() { const [successMessage, setSuccessMessage] = useState(null); const [errorMessage, setErrorMessage] = useState(null); const form = useForm(); const onSubmit = form.handleSubmit(async (data) => { try { const result = await migratePassword(data); setSuccessMessage(result.message); } catch (e) { console.error(e); if (e instanceof Error) { setErrorMessage(e.message); } } }); return ( <div style={{ maxWidth: "400px", margin: "auto", }}> <h1>Migrate your password</h1> <p> If you have an account on the old version of the website, you can migrate your password to the new version. </p> {successMessage && <div>{successMessage}</div>} {errorMessage && <FormError>{errorMessage}</FormError>} <form onSubmit={onSubmit}> <FormItemGroup> <FormLabel>Username</FormLabel> <FormInput {...form.register("username", { required: "Username is required", })} /> <FormError>{form.formState.errors.username?.message}</FormError> </FormItemGroup> <FormItemGroup> <FormLabel>Password</FormLabel> <FormInput {...form.register("password", { required: "Password is required", })} type="password" /> <FormError>{form.formState.errors.password?.message}</FormError> </FormItemGroup> <button type="submit">Migrate password</button> </form> </div> );}
```
3. Finally, you will need to create a new operation in your app to handle the password migration. You can use the following code as a starting point:
* JavaScript
* TypeScript
main.wasp
```
action migratePassword { fn: import { migratePassword } from "@src/auth", entities: []}
```
src/auth.js
```
import SecurePassword from "secure-password";import { HttpError } from "wasp/server";import { createProviderId, deserializeAndSanitizeProviderData, findAuthIdentity, updateAuthIdentityProviderData,} from "wasp/server/auth";export const migratePassword = async ({ password, username }, _context) => { const providerId = createProviderId("username", username); const authIdentity = await findAuthIdentity(providerId); if (!authIdentity) { throw new HttpError(400, "Something went wrong"); } const providerData = deserializeAndSanitizeProviderData( authIdentity.providerData ); try { const SP = new SecurePassword(); // This will verify the password using the old algorithm const result = await SP.verify( Buffer.from(password), Buffer.from(providerData.hashedPassword, "base64") ); if (result !== SecurePassword.VALID) { throw new HttpError(400, "Something went wrong"); } // This will hash the password using the new algorithm and update the // provider data in the database. await updateAuthIdentityProviderData(providerId, providerData, { hashedPassword: password, }); } catch (e) { throw new HttpError(400, "Something went wrong"); } return { message: "Password migrated successfully.", };};
```
#### Email[](#email "Direct link to Email")
To successfully migrate the users using the Email auth method, you will need to do two things:
1. Migrate the user data
Email data migration function
main.wasp
```
api migrateEmail { httpRoute: (GET, "/migrate-email"), fn: import { migrateEmailHandler } from "@src/migrateToNewAuth", entities: []}
```
src/migrateToNewAuth.ts
```
import { prisma } from "wasp/server";import { type ProviderName, type EmailProviderData } from "wasp/server/auth";import { MigrateEmail } from "wasp/server/api";export const migrateEmailHandler: MigrateEmail = async (_req, res) => { const result = await migrateEmailAuth(); res.status(200).json({ message: "Migrated users to the new auth", result }); };async function migrateEmailAuth(): Promise<{ numUsersAlreadyMigrated: number; numUsersNotUsingThisAuthMethod: number; numUsersMigratedSuccessfully: number;}> { const users = await prisma.user.findMany({ include: { auth: true, }, }); const result = { numUsersAlreadyMigrated: 0, numUsersNotUsingThisAuthMethod: 0, numUsersMigratedSuccessfully: 0, }; for (const user of users) { if (user.auth) { result.numUsersAlreadyMigrated++; console.log("Skipping user (already migrated) with id:", user.id); continue; } if (!user.email || !user.password) { result.numUsersNotUsingThisAuthMethod++; console.log("Skipping user (not using email auth) with id:", user.id); continue; } const providerData: EmailProviderData = { isEmailVerified: user.isEmailVerified, emailVerificationSentAt: user.emailVerificationSentAt?.toISOString() ?? null, passwordResetSentAt: user.passwordResetSentAt?.toISOString() ?? null, hashedPassword: user.password, }; const providerName: ProviderName = "email"; await prisma.auth.create({ data: { identities: { create: { providerName, providerUserId: user.email, providerData: JSON.stringify(providerData), }, }, user: { connect: { id: user.id, }, }, }, }); result.numUsersMigratedSuccessfully++; } return result;}
```
2. Ask the users to reset their password
There is a **breaking change between the old and the new auth in the way the password is hashed**. This means that users will need to reset their password after the migration, as the old password will no longer work.
It would be best to notify your users about this change and put a notice on your login page to **request a password reset**.
#### Google & GitHub[](#google--github "Direct link to Google & GitHub")
Google & GitHub data migration functions
main.wasp
```
api migrateGoogle { httpRoute: (GET, "/migrate-google"), fn: import { migrateGoogleHandler } from "@src/migrateToNewAuth", entities: []}api migrateGithub { httpRoute: (GET, "/migrate-github"), fn: import { migrateGithubHandler } from "@src/migrateToNewAuth", entities: []}
```
src/migrateToNewAuth.ts
```
import { prisma } from "wasp/server";import { MigrateGoogle, MigrateGithub } from "wasp/server/api";export const migrateGoogleHandler: MigrateGoogle = async (_req, res) => { const result = await createSocialLoginMigration("google"); res.status(200).json({ message: "Migrated users to the new auth", result }); };export const migrateGithubHandler: MigrateGithub = async (_req, res) => { const result = await createSocialLoginMigration("github"); res.status(200).json({ message: "Migrated users to the new auth", result }); };async function createSocialLoginMigration( providerName: "google" | "github"): Promise<{ numUsersAlreadyMigrated: number; numUsersNotUsingThisAuthMethod: number; numUsersMigratedSuccessfully: number;}> { const users = await prisma.user.findMany({ include: { auth: true, externalAuthAssociations: true, }, }); const result = { numUsersAlreadyMigrated: 0, numUsersNotUsingThisAuthMethod: 0, numUsersMigratedSuccessfully: 0, }; for (const user of users) { if (user.auth) { result.numUsersAlreadyMigrated++; console.log("Skipping user (already migrated) with id:", user.id); continue; } const provider = user.externalAuthAssociations.find( (provider) => provider.provider === providerName ); if (!provider) { result.numUsersNotUsingThisAuthMethod++; console.log(`Skipping user (not using ${providerName} auth) with id:`, user.id); continue; } await prisma.auth.create({ data: { identities: { create: { providerName, providerUserId: provider.providerId, providerData: JSON.stringify({}), }, }, user: { connect: { id: user.id, }, }, }, }); result.numUsersMigratedSuccessfully++; } return result;}
``` | null | https://wasp-lang.dev/docs/0.12.0/migrate-from-0-11-to-0-12 | What's new in Wasp 0.12.0? | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecedaef635b6b-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:16 GMT | Tue, 23 Apr 2024 15:25:16 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=PV9F4TtzybrCHsRji0Fm9%2FR66wl%2FaaNnzirFPgDP6Uf16ycQqof4y3mJumpPW6AQrH70FmrMxEPO1x9aYe5cF6S64dcQGlY4IOcbNBr%2B5DCBTyPvK4M%2FgdRA3MCvJMIX"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | ab4f6863e02aaa75d29ee65a813fd179d29f93cf | null | 1048:218394:3B9E589:46A44F9:6627D084 | HIT | MISS | cache-iad-kiad7000035-IAD | S1713885316.327845,VS0,VE69 | null | null | en | og:image | https://wasp-lang.dev/docs/0.12.0/migrate-from-0-11-to-0-12 | og:url | Migration from 0.11.X to 0.12.X | Wasp | og:title | What's new in Wasp 0.12.0? | og:description | Migration from 0.11.X to 0.12.X | Wasp | null | What's new in Wasp 0.12.0?
New project structure
Here's a file tree of a fresh Wasp project created with the previous version of Wasp. More precisely, this is what you'll get if you run wasp new myProject using Wasp 0.11.x:
.
├── .gitignore
├── main.wasp
├── src
│ ├── client
│ │ ├── Main.css
│ │ ├── MainPage.jsx
│ │ ├── react-app-env.d.ts
│ │ ├── tsconfig.json
│ │ └── waspLogo.png
│ ├── server
│ │ └── tsconfig.json
│ ├── shared
│ │ └── tsconfig.json
│ └── .waspignore
└── .wasproot
Compare that with the file tree of a fresh Wasp project created with Wasp 0.12.0. In other words, this is what you will get by running wasp new myProject from this point onwards:
.
├── .gitignore
├── main.wasp
├── package.json
├── public
│ └── .gitkeep
├── src
│ ├── Main.css
│ ├── MainPage.jsx
│ ├── queries.ts
│ ├── vite-env.d.ts
│ ├── .waspignore
│ └── waspLogo.png
├── tsconfig.json
├── vite.config.ts
└── .wasproot
The main differences are:
The server/client code separation is no longer necessary. You can now organize your code however you want, as long as it's inside the src directory.
All external imports in your Wasp file must have paths starting with @src (e.g., import foo from '@src/bar.js') where @src refers to the src directory in your project root. The paths can no longer start with @server or @client.
Your project now features a top-level public dir. Wasp will publicly serve all the files it finds in this directory. Read more about it here.
Our Overview docs explain the new structure in detail, while this page provides a quick guide for migrating existing projects.
New auth
In Wasp 0.11.X, authentication was based on the User model which the developer needed to set up properly and take care of the auth fields like email or password.
main.wasp
app myApp {
wasp: {
version: "^0.11.0"
},
title: "My App",
auth: {
userEntity: User,
externalAuthEntity: SocialLogin,
methods: {
gitHub: {}
},
onAuthFailedRedirectTo: "/login"
},
}
entity User {=psl
id Int @id @default(autoincrement())
username String @unique
password String
externalAuthAssociations SocialLogin[]
psl=}
entity SocialLogin {=psl
id Int @id @default(autoincrement())
provider String
providerId String
user User @relation(fields: [userId], references: [id], onDelete: Cascade)
userId Int
createdAt DateTime @default(now())
@@unique([provider, providerId, userId])
psl=}
From 0.12.X onwards, authentication is based on the auth models which are automatically set up by Wasp. You don't need to take care of the auth fields anymore.
The User model is now just a business logic model and you use it for storing the data that is relevant for your app.
main.wasp
app myApp {
wasp: {
version: "^0.12.0"
},
title: "My App",
auth: {
userEntity: User,
methods: {
gitHub: {}
},
onAuthFailedRedirectTo: "/login"
},
}
entity User {=psl
id Int @id @default(autoincrement())
psl=}
Regression Note: Multiple Auth Identities per User
With our old auth implementation, if you were using both Google and email auth methods, your users could sign up with Google first and then, later on, reset their password and therefore also enable logging in with their email and password. This was the only way in which a single user could have multiple login methods at the same time (Google and email).
This is not possible anymore. The new auth system doesn't support multiple login methods per user at the moment. We do plan to add this soon though, with the introduction of the account merging feature.
If you have any users that have both Google and email login credentials at the same time, you will have to pick only one of those for that user to keep when migrating them.
Regression Note: _waspCustomValidations is deprecated
Auth field customization is no longer possible using the _waspCustomValidations on the User entity. This is a part of auth refactoring that we are doing to make it easier to customize auth. We will be adding more customization options in the future.
You can read more about the new auth system in the Auth Entities section.
How to Migrate?
These instructions are for migrating your app from Wasp 0.11.X to Wasp 0.12.X, meaning they will work for all minor releases that fit this pattern (e.g., the guide applies to 0.12.0, 0.12.1, ...).
The guide consists of two big steps:
Migrating your Wasp project to the new structure.
Migrating to the new auth.
If you get stuck at any point, don't hesitate to ask for help on our Discord server.
Migrating Your Project to the New Structure
You can easily migrate your old Wasp project to the new structure by following a series of steps. Assuming you have a project called foo inside the directory foo, you should:
Install the latest 0.12.x version of Wasp.
curl -sSL https://get.wasp-lang.dev/installer.sh | sh -s
Make sure to backup or save your project before starting the procedure (e.g., by committing it to source control or creating a copy).
Position yourself in the terminal in the directory that is a parent of your wasp project directory (so one level above: if you do ls, you should see your wasp project dir listed).
Run the migration script (replace foo at the end with the name of your Wasp project directory) and follow the instructions:
In case the migration script doesn't work well for you, you can do the same steps manually, as described here:
Rename your project's root directory to something like foo_old.
Create a new project by running wasp new foo.
Delete all files of foo/src except vite-env.d.ts.
If foo_old/src/client/public exists and contains any files, copy those files into foo/public.
Copy the contents of foo_old/src into foo/src. foo/src should now contain vite-env.d.ts, .waspignore, and three subdirectories (server, client, and shared). Don't change anything about this structure yet.
Delete redundant files and folders from foo/src:
foo/src/.waspignore - A new version of this file already exists at the top level.
foo/src/client/vite-env.d.ts - A new version of this file already exists at the top level.
foo/src/client/tsconfig.json - A new version of this file already exists at the top level.
foo/src/server/tsconfig.json - A new version of this file already exists at the top level.
foo/src/shared/tsconfig.json - A new version of this file already exists at the top level.
foo/src/client/public - You've moved all the files from this directory in step 5.
Update all the @wasp imports in your JS(X)/TS(X) source files in the src/ dir.
For this, we prepared a special script that will rewrite these imports automatically for you.
Before doing this step, as the script will modify your JS(X)/TS(X) files in place, we advise committing all changes you have so far, so you can then both easily inspect the import rewrites that our script did (with git diff) and also revert them if something went wrong.
To run the import-rewriting script, make sure you are in the root dir of your wasp project, and then run
npx [email protected] -t https://raw.githubusercontent.com/wasp-lang/wasp-codemod/main/src/transforms/imports-from-0-11-to-0-12.ts --extensions=js,ts,jsx,tsx src/
Then, check the changes it did, in case some kind of manual intervention is needed (in which case you should see TODO comments generated by the script).
Alternatively, you can find all the mappings of old imports to the new ones in this table and use it to fix some/all of them manually.
Replace the Wasp file in foo (i.e., main.wasp) with the Wasp file from foo_old
Change the Wasp version field in your Wasp file (now residing in foo) to "^0.12.0".
Correct external imports in your Wasp file (now residing in foo). imports. You can do this by running search-and-replace inside the file:
Change all occurrences of @server to @src/server
Change all occurrences of @client to @src/client
For example, if you previously had something like:
page LoginPage {
// This previously resolved to src/client/LoginPage.js
component: import Login from "@client/LoginPage"
}
// ...
query getTasks {
// This previously resolved to src/server/queries.js
fn: import { getTasks } from "@server/queries.js",
}
You should change it to:
page LoginPage {
// This now resolves to src/client/LoginPage.js
component: import Login from "@src/client/LoginPage"
}
// ...
query getTasks {
// This now resolves to src/server/queries.js
fn: import { getTasks } from "@src/server/queries.js",
}
Do this for all external imports in your .wasp file. After you're done, there shouldn't be any occurrences of strings "@server" or "@client"
Take all the dependencies from app.dependencies declaration in foo/main.wasp and move them to foo/package.json. Make sure to remove the app.dependencies field from foo/main.wasp.
For example, if foo_old/main.wasp had:
app Foo {
// ...
dependencies: [ ('redux', '^4.0.5'), ('reacjt-redux', '^7.1.3')];
}
Your package.json in foo should now list these dependencies (Wasp already generated most of the file, you just have to list additional dependencies).
{
"name": "foo",
"dependencies": {
"wasp": "file:.wasp/out/sdk/wasp",
"react": "^18.2.0",
"redux": "^4.0.5",
"reactjs-redux": "^7.1.3"
},
"devDependencies": {
"typescript": "^5.1.0",
"vite": "^4.3.9",
"@types/react": "^18.0.37",
"prisma": "4.16.2"
}
}
Copy all lines you might have added to foo_old/.gitignore into foo/.gitignore
Copy the rest of the top-level files and folders (all of them except for .gitignore, main.wasp and src/) in foo_old/ into foo/ (overwrite the existing files in foo).
Run wasp clean in foo.
Delete the foo_old directory.
That's it! You now have a properly structured Wasp 0.12.0 project in the foo directory. Your app probably doesn't quite work yet due to some other changes in Wasp 0.12.0, but we'll get to that in the next sections.
Migrating declaration names
Wasp 0.12.0 adds a casing constraints when naming Queries, Actions, Jobs, and Entities in the main.wasp file.
The following casing conventions have now become mandatory:
Operation (i.e., Query and Action) names must begin with a lowercase letter: query getTasks {...}, action createTask {...}.
Job names must begin with a lowercase letter: job sendReport {...}.
Entity names must start with an uppercase letter: entity Task {...}.
Migrating the Tailwind Setup
note
If you don't use Tailwind in your project, you can skip this section.
There is a small change in how the tailwind.config.cjs needs to be defined in Wasp 0.12.0.
You'll need to wrap all your paths in the content field with the resolveProjectPath function. This makes sure that the paths are resolved correctly when generating your CSS.
Here's how you can do it:
Before
After
tailwind.config.cjs
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
'./src/**/*.{js,jsx,ts,tsx}',
],
theme: {
extend: {},
},
plugins: [],
}
Default Server Dockerfile Changed
note
If you didn't customize your Dockerfile or had a custom build process for the Wasp server, you can skip this section.
Between Wasp 0.11.X and 0.12.X, the Dockerfile that Wasp generates for you for deploying the server has changed. If you defined a custom Dockerfile in your project root dir or in any other way relied on its contents, you'll need to update it to incorporate the changes that Wasp 0.12.X made.
We suggest that you temporarily move your custom Dockerfile to a different location, then run wasp start to generate the new Dockerfile. Check out the .wasp/out/Dockerfile to see the new Dockerfile and what changes you need to make. You'll probably need to copy some of the changes from the new Dockerfile to your custom one to make your app work with Wasp 0.12.X.
Migrating to the New Auth
As shown in the previous section, Wasp significantly changed how authentication works in version 0.12.0. This section leads you through migrating your app from Wasp 0.11.X to Wasp 0.12.X.
Migrating your existing app to the new auth system is a two-step process:
Migrate to the new auth system
Clean up the old auth system
Migrating a deployed app
While going through these steps, we will focus first on doing the changes locally (including your local development database).
Once we confirm everything works well locally, we will apply the same changes to the deployed app (including your production database).
We'll put extra info for migrating a deployed app in a box like this one.
1. Migrate to the New Auth System
You can follow these steps to migrate to the new auth system (assuming you already migrated the project structure to 0.12, as described above):
Migrate getUserFields and/or additionalSignupFields in the main.wasp file to the new userSignupFields field.
If you are not using them, you can skip this step.
In Wasp 0.11.X, you could define a getUserFieldsFn to specify extra fields that would get saved to the User when using Google or GitHub to sign up.
You could also define additionalSignupFields to specify extra fields for the Email or Username & Password signup.
In 0.12.X, we unified these two concepts into the userSignupFields field.
Migration for Email and Username & Password
First, move the value of auth.signup.additionalFields to auth.methods.{method}.userSignupFields in the main.wasp file.
{method} depends on the auth method you are using. For example, if you are using the email auth method, you should move the auth.signup.additionalFields to auth.methods.email.userSignupFields.
To finish, update the JS/TS implementation to use the defineUserSignupFields from wasp/server/auth instead of defineAdditionalSignupFields from @wasp/auth/index.js.
Before
After
main.wasp
app crudTesting {
// ...
auth: {
userEntity: User,
methods: {
email: {},
},
onAuthFailedRedirectTo: "/login",
signup: {
additionalFields: import { fields } from "@server/auth/signup.js",
},
},
}
src/server/auth/signup.ts
import { defineAdditionalSignupFields } from '@wasp/auth/index.js'
export const fields = defineAdditionalSignupFields({
address: async (data) => {
const address = data.address
if (typeof address !== 'string') {
throw new Error('Address is required')
}
if (address.length < 5) {
throw new Error('Address must be at least 5 characters long')
}
return address
},
})
Migration for Github and Google
First, move the value of auth.methods.{method}.getUserFieldsFn to auth.methods.{method}.userSignupFields in the main.wasp file.
{method} depends on the auth method you are using. For example, if you are using Google auth, you should move the auth.methods.google.getUserFieldsFn to auth.methods.google.userSignupFields.
To finish, update the JS/TS implementation to use the defineUserSignupFields from wasp/server/auth and modify the code to return the fields in the format that defineUserSignupFields expects.
Before
After
main.wasp
app crudTesting {
// ...
auth: {
userEntity: User,
methods: {
google: {
getUserFieldsFn: import { getUserFields } from "@server/auth/google.js"
},
},
onAuthFailedRedirectTo: "/login",
},
}
src/server/auth/google.ts
import type { GetUserFieldsFn } from '@wasp/types'
export const getUserFields: GetUserFieldsFn = async (_context, args) => {
const displayName = args.profile.displayName
return { displayName }
}
Remove the auth.methods.email.allowUnverifiedLogin field from your main.wasp file.
In Wasp 0.12.X we removed the auth.methods.email.allowUnverifiedLogin field to make our Email auth implementation easier to reason about. If you were using it, you should remove it from your main.wasp file.
Ensure your local development database is running.
Do the schema migration (create the new auth tables in the database) by running:
You should see the new Auth, AuthIdentity and Session tables in your database. You can use the wasp db studio command to open the database in a GUI and verify the tables are there. At the moment, they will be empty.
Do the data migration (move existing users from the old auth system to the new one by filling the new auth tables in the database with their data):
Implement your data migration function(s) in e.g. src/migrateToNewAuth.ts.
Below we prepared examples of migration functions for each of the auth methods, for you to use as a starting point. They should be fine to use as-is, meaning you can just copy them and they are likely to work out of the box for typical use cases, but you can also modify them for your needs.
We recommend you create one function per each auth method that you use in your app.
Define custom API endpoints for each migration function you implemented.
With each data migration function below, we provided a relevant api declaration that you should add to your main.wasp file.
Run the data migration function(s) on the local development database by calling the API endpoints you defined in the previous step.
You can call the endpoint by visiting the URL in your browser, or by using a tool like curl or Postman.
For example, if you defined the API endpoint at /migrate-username-and-password, you can call it by visiting http://localhost:3001/migrate-username-and-password in your browser.
This should be it, you can now run wasp db studio again and verify that there is now relevant data in the new auth tables (Auth and AuthIdentity; Session should still be empty for now).
Verify that the basic auth functionality works by running wasp start and successfully signing up / logging in with each of the auth methods.
Update your JS/TS code to work correctly with the new auth.
You might want to use the new auth helper functions to get the email or username from a user object. For example, user.username might not work anymore for you, since the username obtained by the Username & Password auth method isn't stored on the User entity anymore (unless you are explicitly storing something into user.username, e.g. via userSignupFields for a social auth method like Github). Same goes for email from Email auth method.
Instead, you can now use getUsername(user) to get the username obtained from Username & Password auth method, or getEmail(user) to get the email obtained from Email auth method.
Read more about the helpers in the Auth Entities - Accessing the Auth Fields section.
Finally, check that your app now fully works as it worked before. If all the above steps were done correctly, everything should be working now.
Migrating a deployed app
After successfully performing migration locally so far, and verifying that your app works as expected, it is time to also migrate our deployed app.
Before migrating your production (deployed) app, we advise you to back up your production database in case something goes wrong. Also, besides testing it in development, it's good to test the migration in a staging environment if you have one.
We will perform the production migration in 2 steps:
Deploying the new code to production (client and server).
Migrating the production database data.
Between these two steps, so after successfully deploying the new code to production and before migrating the production database data, your app will not be working completely: new users will be able to sign up, but existing users won't be able to log in, and already logged in users will be logged out. Once you do the second step, migrating the production database data, it will all be back to normal. You will likely want to keep the time between the two steps as short as you can.
First step: deploy the new code (client and server), either via wasp deploy (i.e. wasp deploy fly deploy) or manually.
Check our Deployment docs for more details.
Second step: run the data migration functions on the production database.
You can do this by calling the API endpoints you defined in the previous step, just like you did locally. You can call the endpoint by visiting the URL in your browser, or by using a tool like curl or Postman.
For example, if you defined the API endpoint at /migrate-username-and-password, you can call it by visiting https://your-server-url.com/migrate-username-and-password in your browser.
Your deployed app should be working normally now, with the new auth system.
2. Cleanup the Old Auth System
Your app should be working correctly and using new auth, but to finish the migration, we need to clean up the old auth system:
In main.wasp file, delete auth-related fields from the User entity, since with 0.12 they got moved to the internal Wasp entity AuthIdentity.
This means any fields that were required by Wasp for authentication, like email, password, isEmailVerified, emailVerificationSentAt, passwordResetSentAt, username, etc.
There are situations in which you might want to keep some of them, e.g. email and/or username, if they are still relevant for you due to your custom logic (e.g. you are populating them with userSignupFields upon social signup in order to have this info easily available on the User entity). Note that they won't be used by Wasp Auth anymore, they are here just for your business logic.
In main.wasp file, remove the externalAuthEntity field from the app.auth and also remove the whole SocialLogin entity if you used Google or GitHub auth.
Delete the data migration function(s) you implemented earlier (e.g. in src/migrateToNewAuth.ts) and also the corresponding API endpoints from the main.wasp file.
Run wasp db migrate-dev again to apply these changes and remove the redundant fields from the database.
Migrating a deployed app
After doing the steps above successfully locally and making sure everything is working, it is time to push these changes to the deployed app again.
Deploy the app again, either via wasp deploy or manually. Check our Deployment docs for more details.
The database migrations will automatically run on successful deployment of the server and delete the now redundant auth-related User columns from the database.
Your app is now fully migrated to the new auth system.
Next Steps
If you made it this far, you've completed all the necessary steps to get your Wasp app working with Wasp 0.12.x. Nice work!
Finally, since Wasp no longer requires you to separate your client source files (previously in src/client) from server source files (previously in src/server), you are now free to reorganize your project however you think is best, as long as you keep all the source files in the src/ directory.
This section is optional, but if you didn't like the server/client separation, now's the perfect time to change it.
For example, if your src dir looked like this:
src
│
├── client
│ ├── Dashboard.tsx
│ ├── Login.tsx
│ ├── MainPage.tsx
│ ├── Register.tsx
│ ├── Task.css
│ ├── TaskLisk.tsx
│ ├── Task.tsx
│ └── User.tsx
├── server
│ ├── taskActions.ts
│ ├── taskQueries.ts
│ ├── userActions.ts
│ └── userQueries.ts
└── shared
└── utils.ts
you can now change it to a feature-based structure (which we recommend for any project that is not very small):
src
│
├── task
│ ├── actions.ts -- former taskActions.ts
│ ├── queries.ts -- former taskQueries.ts
│ ├── Task.css
│ ├── TaskLisk.tsx
│ └── Task.tsx
├── user
│ ├── actions.ts -- former userActions.ts
│ ├── Dashboard.tsx
│ ├── Login.tsx
│ ├── queries.ts -- former userQueries.ts
│ ├── Register.tsx
│ └── User.tsx
├── MainPage.tsx
└── utils.ts
Appendix
Example Data Migration Functions
The migration functions provided below are written with the typical use cases in mind and you can use them as-is. If your setup requires additional logic, you can use them as a good starting point and modify them to your needs.
Note that all of the functions below are written to be idempotent, meaning that running a function multiple times can't hurt. This allows executing a function again in case only a part of the previous execution succeeded and also means that accidentally running it one time too much won't have any negative effects. We recommend you keep your data migration functions idempotent.
Username & Password
To successfully migrate the users using the Username & Password auth method, you will need to do two things:
Migrate the user data
Username & Password data migration function
main.wasp
api migrateUsernameAndPassword {
httpRoute: (GET, "/migrate-username-and-password"),
fn: import { migrateUsernameAndPasswordHandler } from "@src/migrateToNewAuth",
entities: []
}
src/migrateToNewAuth.ts
import { prisma } from "wasp/server";
import { type ProviderName, type UsernameProviderData } from "wasp/server/auth";
import { MigrateUsernameAndPassword } from "wasp/server/api";
export const migrateUsernameAndPasswordHandler: MigrateUsernameAndPassword =
async (_req, res) => {
const result = await migrateUsernameAuth();
res.status(200).json({ message: "Migrated users to the new auth", result });
};
async function migrateUsernameAuth(): Promise<{
numUsersAlreadyMigrated: number;
numUsersNotUsingThisAuthMethod: number;
numUsersMigratedSuccessfully: number;
}> {
const users = await prisma.user.findMany({
include: {
auth: true,
},
});
const result = {
numUsersAlreadyMigrated: 0,
numUsersNotUsingThisAuthMethod: 0,
numUsersMigratedSuccessfully: 0,
};
for (const user of users) {
if (user.auth) {
result.numUsersAlreadyMigrated++;
console.log("Skipping user (already migrated) with id:", user.id);
continue;
}
if (!user.username || !user.password) {
result.numUsersNotUsingThisAuthMethod++;
console.log("Skipping user (not using username auth) with id:", user.id);
continue;
}
const providerData: UsernameProviderData = {
hashedPassword: user.password,
};
const providerName: ProviderName = "username";
await prisma.auth.create({
data: {
identities: {
create: {
providerName,
providerUserId: user.username.toLowerCase(),
providerData: JSON.stringify(providerData),
},
},
user: {
connect: {
id: user.id,
},
},
},
});
result.numUsersMigratedSuccessfully++;
}
return result;
}
Provide a way for users to migrate their password
There is a breaking change between the old and the new auth in the way the password is hashed. This means that users will need to migrate their password after the migration, as the old password will no longer work.
Since the only way users using username and password as a login method can verify their identity is by providing both their username and password (there is no email or any other info, unless you asked for it and stored it explicitly), we need to provide them a way to exchange their old password for a new password. One way to handle this is to inform them about the need to migrate their password (on the login page) and provide a custom page to migrate the password.
Steps to create a custom page for migrating the password
You will need to install the secure-password and sodium-native packages to use the old hashing algorithm:
Make sure to save the exact versions of the packages.
Then you'll need to create a new page in your app where users can migrate their password. You can use the following code as a starting point:
JavaScript
TypeScript
main.wasp
route MigratePasswordRoute { path: "/migrate-password", to: MigratePassword }
page MigratePassword {
component: import { MigratePasswordPage } from "@src/pages/MigratePassword"
}
src/pages/MigratePassword.jsx
import {
FormItemGroup,
FormLabel,
FormInput,
FormError,
} from "wasp/client/auth";
import { useForm } from "react-hook-form";
import { migratePassword } from "wasp/client/operations";
import { useState } from "react";
export function MigratePasswordPage() {
const [successMessage, setSuccessMessage] = useState(null);
const [errorMessage, setErrorMessage] = useState(null);
const form = useForm();
const onSubmit = form.handleSubmit(async (data) => {
try {
const result = await migratePassword(data);
setSuccessMessage(result.message);
} catch (e) {
console.error(e);
if (e instanceof Error) {
setErrorMessage(e.message);
}
}
});
return (
<div style={{
maxWidth: "400px",
margin: "auto",
}}>
<h1>Migrate your password</h1>
<p>
If you have an account on the old version of the website, you can
migrate your password to the new version.
</p>
{successMessage && <div>{successMessage}</div>}
{errorMessage && <FormError>{errorMessage}</FormError>}
<form onSubmit={onSubmit}>
<FormItemGroup>
<FormLabel>Username</FormLabel>
<FormInput
{...form.register("username", {
required: "Username is required",
})}
/>
<FormError>{form.formState.errors.username?.message}</FormError>
</FormItemGroup>
<FormItemGroup>
<FormLabel>Password</FormLabel>
<FormInput
{...form.register("password", {
required: "Password is required",
})}
type="password"
/>
<FormError>{form.formState.errors.password?.message}</FormError>
</FormItemGroup>
<button type="submit">Migrate password</button>
</form>
</div>
);
}
Finally, you will need to create a new operation in your app to handle the password migration. You can use the following code as a starting point:
JavaScript
TypeScript
main.wasp
action migratePassword {
fn: import { migratePassword } from "@src/auth",
entities: []
}
src/auth.js
import SecurePassword from "secure-password";
import { HttpError } from "wasp/server";
import {
createProviderId,
deserializeAndSanitizeProviderData,
findAuthIdentity,
updateAuthIdentityProviderData,
} from "wasp/server/auth";
export const migratePassword = async ({ password, username }, _context) => {
const providerId = createProviderId("username", username);
const authIdentity = await findAuthIdentity(providerId);
if (!authIdentity) {
throw new HttpError(400, "Something went wrong");
}
const providerData = deserializeAndSanitizeProviderData(
authIdentity.providerData
);
try {
const SP = new SecurePassword();
// This will verify the password using the old algorithm
const result = await SP.verify(
Buffer.from(password),
Buffer.from(providerData.hashedPassword, "base64")
);
if (result !== SecurePassword.VALID) {
throw new HttpError(400, "Something went wrong");
}
// This will hash the password using the new algorithm and update the
// provider data in the database.
await updateAuthIdentityProviderData(providerId, providerData, {
hashedPassword: password,
});
} catch (e) {
throw new HttpError(400, "Something went wrong");
}
return {
message: "Password migrated successfully.",
};
};
Email
To successfully migrate the users using the Email auth method, you will need to do two things:
Migrate the user data
Email data migration function
main.wasp
api migrateEmail {
httpRoute: (GET, "/migrate-email"),
fn: import { migrateEmailHandler } from "@src/migrateToNewAuth",
entities: []
}
src/migrateToNewAuth.ts
import { prisma } from "wasp/server";
import { type ProviderName, type EmailProviderData } from "wasp/server/auth";
import { MigrateEmail } from "wasp/server/api";
export const migrateEmailHandler: MigrateEmail =
async (_req, res) => {
const result = await migrateEmailAuth();
res.status(200).json({ message: "Migrated users to the new auth", result });
};
async function migrateEmailAuth(): Promise<{
numUsersAlreadyMigrated: number;
numUsersNotUsingThisAuthMethod: number;
numUsersMigratedSuccessfully: number;
}> {
const users = await prisma.user.findMany({
include: {
auth: true,
},
});
const result = {
numUsersAlreadyMigrated: 0,
numUsersNotUsingThisAuthMethod: 0,
numUsersMigratedSuccessfully: 0,
};
for (const user of users) {
if (user.auth) {
result.numUsersAlreadyMigrated++;
console.log("Skipping user (already migrated) with id:", user.id);
continue;
}
if (!user.email || !user.password) {
result.numUsersNotUsingThisAuthMethod++;
console.log("Skipping user (not using email auth) with id:", user.id);
continue;
}
const providerData: EmailProviderData = {
isEmailVerified: user.isEmailVerified,
emailVerificationSentAt:
user.emailVerificationSentAt?.toISOString() ?? null,
passwordResetSentAt: user.passwordResetSentAt?.toISOString() ?? null,
hashedPassword: user.password,
};
const providerName: ProviderName = "email";
await prisma.auth.create({
data: {
identities: {
create: {
providerName,
providerUserId: user.email,
providerData: JSON.stringify(providerData),
},
},
user: {
connect: {
id: user.id,
},
},
},
});
result.numUsersMigratedSuccessfully++;
}
return result;
}
Ask the users to reset their password
There is a breaking change between the old and the new auth in the way the password is hashed. This means that users will need to reset their password after the migration, as the old password will no longer work.
It would be best to notify your users about this change and put a notice on your login page to request a password reset.
Google & GitHub
Google & GitHub data migration functions
main.wasp
api migrateGoogle {
httpRoute: (GET, "/migrate-google"),
fn: import { migrateGoogleHandler } from "@src/migrateToNewAuth",
entities: []
}
api migrateGithub {
httpRoute: (GET, "/migrate-github"),
fn: import { migrateGithubHandler } from "@src/migrateToNewAuth",
entities: []
}
src/migrateToNewAuth.ts
import { prisma } from "wasp/server";
import { MigrateGoogle, MigrateGithub } from "wasp/server/api";
export const migrateGoogleHandler: MigrateGoogle =
async (_req, res) => {
const result = await createSocialLoginMigration("google");
res.status(200).json({ message: "Migrated users to the new auth", result });
};
export const migrateGithubHandler: MigrateGithub =
async (_req, res) => {
const result = await createSocialLoginMigration("github");
res.status(200).json({ message: "Migrated users to the new auth", result });
};
async function createSocialLoginMigration(
providerName: "google" | "github"
): Promise<{
numUsersAlreadyMigrated: number;
numUsersNotUsingThisAuthMethod: number;
numUsersMigratedSuccessfully: number;
}> {
const users = await prisma.user.findMany({
include: {
auth: true,
externalAuthAssociations: true,
},
});
const result = {
numUsersAlreadyMigrated: 0,
numUsersNotUsingThisAuthMethod: 0,
numUsersMigratedSuccessfully: 0,
};
for (const user of users) {
if (user.auth) {
result.numUsersAlreadyMigrated++;
console.log("Skipping user (already migrated) with id:", user.id);
continue;
}
const provider = user.externalAuthAssociations.find(
(provider) => provider.provider === providerName
);
if (!provider) {
result.numUsersNotUsingThisAuthMethod++;
console.log(`Skipping user (not using ${providerName} auth) with id:`, user.id);
continue;
}
await prisma.auth.create({
data: {
identities: {
create: {
providerName,
providerUserId: provider.providerId,
providerData: JSON.stringify({}),
},
},
user: {
connect: {
id: user.id,
},
},
},
});
result.numUsersMigratedSuccessfully++;
}
return result;
} | https://wasp-lang.dev/docs/0.12.0/migrate-from-0-11-to-0-12 |
|
2 | null | 2024-04-23T15:15:17.430Z | https://wasp-lang.dev/docs/0.11.8/project/server-config | https://wasp-lang.dev/docs/0.11.8 | http | ## Server Config
You can configure the behavior of the server via the `server` field of `app` declaration:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { title: "My app", // ... server: { setupFn: import { mySetupFunction } from "@server/myServerSetupCode.js", middlewareConfigFn: import { myMiddlewareConfigFn } from "@server/myServerSetupCode.js" }}
```
## Setup Function[](#setup-function "Direct link to Setup Function")
### Adding a Custom Route[](#adding-a-custom-route "Direct link to Adding a Custom Route")
As an example, adding a custom route would look something like:
* JavaScript
* TypeScript
src/server/myServerSetupCode.ts
```
export const mySetupFunction = async ({ app }) => { addCustomRoute(app)}function addCustomRoute(app) { app.get('/customRoute', (_req, res) => { res.send('I am a custom route') })}
```
### Storing Some Values for Later Use[](#storing-some-values-for-later-use "Direct link to Storing Some Values for Later Use")
In case you want to store some values for later use, or to be accessed by the [Operations](https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview) you do that in the `setupFn` function.
Dummy example of such function and its usage:
* JavaScript
* TypeScript
src/server/myServerSetupCode.js
```
let someResource = undefinedexport const mySetupFunction = async () => { // Let's pretend functions setUpSomeResource and startSomeCronJob // are implemented below or imported from another file. someResource = await setUpSomeResource() startSomeCronJob()}export const getSomeResource = () => someResource
```
src/server/queries.js
```
import { getSomeResource } from './myServerSetupCode.js'...export const someQuery = async (args, context) => { const someResource = getSomeResource() return queryDataFromSomeResource(args, someResource)}
```
note
The recommended way is to put the variable in the same module where you defined the setup function and then expose additional functions for reading those values, which you can then import directly from Operations and use.
This effectively turns your module into a singleton whose construction is performed on server start.
Read more about [server setup function](#setupfn-serverimport) below.
## Middleware Config Function[](#middleware-config-function "Direct link to Middleware Config Function")
You can configure the global middleware via the `middlewareConfigFn`. This will modify the middleware stack for all operations and APIs.
Read more about [middleware config function](#middlewareconfigfn-serverimport) below.
## API Reference[](#api-reference "Direct link to API Reference")
* JavaScript
* TypeScript
main.wasp
```
app MyApp { title: "My app", // ... server: { setupFn: import { mySetupFunction } from "@server/myServerSetupCode.js", middlewareConfigFn: import { myMiddlewareConfigFn } from "@server/myServerSetupCode.js" }}
```
`app.server` is a dictionary with the following fields:
* #### `setupFn: ServerImport`[](#setupfn-serverimport "Direct link to setupfn-serverimport")
`setupFn` declares a function that will be executed on server start. This function is expected to be async and will be awaited before the server starts accepting any requests.
It allows you to do any custom setup, e.g. setting up additional database/websockets or starting cron/scheduled jobs.
The `setupFn` function receives the `express.Application` and the `http.Server` instances as part of its context. They can be useful for setting up any custom server routes or for example, setting up `socket.io`.
* JavaScript
* TypeScript
src/server/myServerSetupCode.js
```
export const mySetupFunction = async () => { await setUpSomeResource()}
```
* #### `middlewareConfigFn: ServerImport`[](#middlewareconfigfn-serverimport "Direct link to middlewareconfigfn-serverimport")
The import statement to an Express middleware config function. This is a global modification affecting all operations and APIs. See more in the [configuring middleware section](https://wasp-lang.dev/docs/0.11.8/advanced/middleware-config#1-customize-global-middleware). | null | https://wasp-lang.dev/docs/0.11.8/project/server-config | You can configure the behavior of the server via the server field of app declaration: | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecee1280907b0-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:17 GMT | Tue, 23 Apr 2024 15:25:17 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=oesYDBlc3R0RNMr9Ejn1hxNbxZJtWaMFwJIiDzM2aKtpY8PWgumuvpmzSx%2FME5tHZ4OoTfHEZ3tmPyGDBrYOez0XgsyC0Vr90DFKcKAXEYdek68tNigKpeaGosSuZz7%2B"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 732f7c4d1165185a991ccba3ec6102961505f9e0 | null | 2FC4:16D7:486383:557B45:6627D084 | HIT | MISS | cache-iad-kiad7000119-IAD | S1713885317.351052,VS0,VE44 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/project/server-config | og:url | Server Config | Wasp | og:title | You can configure the behavior of the server via the server field of app declaration: | og:description | Server Config | Wasp | null | Server Config
You can configure the behavior of the server via the server field of app declaration:
JavaScript
TypeScript
main.wasp
app MyApp {
title: "My app",
// ...
server: {
setupFn: import { mySetupFunction } from "@server/myServerSetupCode.js",
middlewareConfigFn: import { myMiddlewareConfigFn } from "@server/myServerSetupCode.js"
}
}
Setup Function
Adding a Custom Route
As an example, adding a custom route would look something like:
JavaScript
TypeScript
src/server/myServerSetupCode.ts
export const mySetupFunction = async ({ app }) => {
addCustomRoute(app)
}
function addCustomRoute(app) {
app.get('/customRoute', (_req, res) => {
res.send('I am a custom route')
})
}
Storing Some Values for Later Use
In case you want to store some values for later use, or to be accessed by the Operations you do that in the setupFn function.
Dummy example of such function and its usage:
JavaScript
TypeScript
src/server/myServerSetupCode.js
let someResource = undefined
export const mySetupFunction = async () => {
// Let's pretend functions setUpSomeResource and startSomeCronJob
// are implemented below or imported from another file.
someResource = await setUpSomeResource()
startSomeCronJob()
}
export const getSomeResource = () => someResource
src/server/queries.js
import { getSomeResource } from './myServerSetupCode.js'
...
export const someQuery = async (args, context) => {
const someResource = getSomeResource()
return queryDataFromSomeResource(args, someResource)
}
note
The recommended way is to put the variable in the same module where you defined the setup function and then expose additional functions for reading those values, which you can then import directly from Operations and use.
This effectively turns your module into a singleton whose construction is performed on server start.
Read more about server setup function below.
Middleware Config Function
You can configure the global middleware via the middlewareConfigFn. This will modify the middleware stack for all operations and APIs.
Read more about middleware config function below.
API Reference
JavaScript
TypeScript
main.wasp
app MyApp {
title: "My app",
// ...
server: {
setupFn: import { mySetupFunction } from "@server/myServerSetupCode.js",
middlewareConfigFn: import { myMiddlewareConfigFn } from "@server/myServerSetupCode.js"
}
}
app.server is a dictionary with the following fields:
setupFn: ServerImport
setupFn declares a function that will be executed on server start. This function is expected to be async and will be awaited before the server starts accepting any requests.
It allows you to do any custom setup, e.g. setting up additional database/websockets or starting cron/scheduled jobs.
The setupFn function receives the express.Application and the http.Server instances as part of its context. They can be useful for setting up any custom server routes or for example, setting up socket.io.
JavaScript
TypeScript
src/server/myServerSetupCode.js
export const mySetupFunction = async () => {
await setUpSomeResource()
}
middlewareConfigFn: ServerImport
The import statement to an Express middleware config function. This is a global modification affecting all operations and APIs. See more in the configuring middleware section. | https://wasp-lang.dev/docs/0.11.8/project/server-config |
|
2 | null | 2024-04-23T15:15:20.241Z | https://wasp-lang.dev/docs/0.11.8/project/env-vars | https://wasp-lang.dev/docs/0.11.8 | http | ## Env Variables
**Environment variables** are used to configure projects based on the context in which they run. This allows them to exhibit different behaviors in different environments, such as development, staging, or production.
For instance, _during development_, you may want your project to connect to a local development database running on your machine, but _in production_, you may prefer it to connect to the production database. Similarly, in development, you may want to use a test Stripe account, while in production, your app should use a real Stripe account.
While some env vars are required by Wasp, such as the database connection or secrets for social auth, you can also define your env vars for any other useful purposes.
In Wasp, you can use environment variables in both the client and the server code.
## Client Env Vars[](#client-env-vars "Direct link to Client Env Vars")
Client environment variables are embedded into the client code during the build and shipping process, making them public and readable by anyone. Therefore, you should **never store secrets in them** (such as secret API keys).
To enable Wasp to pick them up, client environment variables must be prefixed with `REACT_APP_`, for example: `REACT_APP_SOME_VAR_NAME=...`.
You can read them from the client code like this:
* JavaScript
* TypeScript
src/App.js
```
console.log(import.meta.env.REACT_APP_SOME_VAR_NAME)
```
Check below on how to define them.
## Server Env Vars[](#server-env-vars "Direct link to Server Env Vars")
In server environment variables, you can store secret values (e.g. secret API keys) since are not publicly readable. You can define them without any special prefix, such as `SOME_VAR_NAME=...`.
You can read them in the server code like this:
* JavaScript
* TypeScript
```
console.log(process.env.SOME_VAR_NAME)
```
Check below on how to define them.
## Defining Env Vars in Development[](#defining-env-vars-in-development "Direct link to Defining Env Vars in Development")
During development, there are two ways to provide env vars to your Wasp project:
1. Using `.env` files. **(recommended)**
2. Using shell. (useful for overrides)
### 1\. Using .env (dotenv) Files[](#1-using-env-dotenv-files "Direct link to 1. Using .env (dotenv) Files")

This is the recommended method for providing env vars to your Wasp project during development.
In the root of your Wasp project you can create two distinct files:
* `.env.server` for env vars that will be provided to the server.
Variables are defined in these files in the form of `NAME=VALUE`, for example:
.env.server
```
DATABASE_URL=postgresql://localhost:5432SOME_VAR_NAME=somevalue
```
* `.env.client` for env vars that will be provided to the client.
Variables are defined in these files in the form of `NAME=VALUE`, for example:
.env.client
```
REACT_APP_SOME_VAR_NAME=somevalue
```
These files should not be committed to version control, and they are already ignored by default in the `.gitignore` file that comes with Wasp.
### 2\. Using Shell[](#2-using-shell "Direct link to 2. Using Shell")
If you set environment variables in the shell where you run your Wasp commands (e.g., `wasp start`), Wasp will recognize them.
You can set environment variables in the `.profile` or a similar file, or by defining them at the start of a command:
```
SOME_VAR_NAME=SOMEVALUE wasp start
```
This is not specific to Wasp and is simply how environment variables can be set in the shell.
Defining environment variables in this way can be cumbersome even for a single project and even more challenging to manage if you have multiple Wasp projects. Therefore, we do not recommend this as a default method for providing environment variables to Wasp projects. However, it can be useful for occasionally **overriding** specific environment variables because environment variables set this way **take precedence over those defined in `.env` files**.
## Defining Env Vars in Production[](#defining-env-vars-in-production "Direct link to Defining Env Vars in Production")
While in development, we had the option of using `.env` files which made it easy to define and manage env vars. However, in production, we need to provide env vars differently.

### Client Env Vars[](#client-env-vars-1 "Direct link to Client Env Vars")
Client env vars are embedded into the client code during the build and shipping process, making them public and readable by anyone. Therefore, you should **never store secrets in them** (such as secret API keys).
You should provide them to the build command, for example:
```
REACT_APP_SOME_VAR_NAME=somevalue npm run build
```
How it works
What happens behind the scenes is that Wasp will replace all occurrences of `import.meta.env.REACT_APP_SOME_VAR_NAME` with the value you provided. This is done during the build process, so the value is embedded into the client code.
Read more about it in Vite's [docs](https://vitejs.dev/guide/env-and-mode.html#production-replacement).
### Server Env Vars[](#server-env-vars-1 "Direct link to Server Env Vars")
The way you provide env vars to your Wasp project in production depends on where you deploy it. For example, if you deploy your project to [Fly](https://fly.io/), you can define them using the `flyctl` CLI tool:
```
flyctl secrets set SOME_VAR_NAME=somevalue
```
You can read a lot more details in the [deployment section](https://wasp-lang.dev/docs/0.11.8/advanced/deployment/manually) of the docs. We go into detail on how to define env vars for each deployment option. | null | https://wasp-lang.dev/docs/0.11.8/project/env-vars | Environment variables are used to configure projects based on the context in which they run. This allows them to exhibit different behaviors in different environments, such as development, staging, or production. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecef31ab21fdc-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:20 GMT | Tue, 23 Apr 2024 15:25:20 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=rBgA2PuG%2BSbnKVo64yAeZwKfQpIc3YYnPpLl9ZFiBNmTcZWIR0fnU70%2FFM0WRdF%2BHl63qtWrSXgYZSbquAbfmrEzgamSnuqt%2FRRYyd%2BAxaF0Xog%2Fw3RoL2bnzmueawNL"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | b3e5213ae69dfd7371665140dfcc448ae1b2b9dc | null | C754:16C4F4:390F24E:441535A:6627D087 | HIT | MISS | cache-iad-kiad7000113-IAD | S1713885320.204356,VS0,VE9 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/project/env-vars | og:url | Env Variables | Wasp | og:title | Environment variables are used to configure projects based on the context in which they run. This allows them to exhibit different behaviors in different environments, such as development, staging, or production. | og:description | Env Variables | Wasp | null | Env Variables
Environment variables are used to configure projects based on the context in which they run. This allows them to exhibit different behaviors in different environments, such as development, staging, or production.
For instance, during development, you may want your project to connect to a local development database running on your machine, but in production, you may prefer it to connect to the production database. Similarly, in development, you may want to use a test Stripe account, while in production, your app should use a real Stripe account.
While some env vars are required by Wasp, such as the database connection or secrets for social auth, you can also define your env vars for any other useful purposes.
In Wasp, you can use environment variables in both the client and the server code.
Client Env Vars
Client environment variables are embedded into the client code during the build and shipping process, making them public and readable by anyone. Therefore, you should never store secrets in them (such as secret API keys).
To enable Wasp to pick them up, client environment variables must be prefixed with REACT_APP_, for example: REACT_APP_SOME_VAR_NAME=....
You can read them from the client code like this:
JavaScript
TypeScript
src/App.js
console.log(import.meta.env.REACT_APP_SOME_VAR_NAME)
Check below on how to define them.
Server Env Vars
In server environment variables, you can store secret values (e.g. secret API keys) since are not publicly readable. You can define them without any special prefix, such as SOME_VAR_NAME=....
You can read them in the server code like this:
JavaScript
TypeScript
console.log(process.env.SOME_VAR_NAME)
Check below on how to define them.
Defining Env Vars in Development
During development, there are two ways to provide env vars to your Wasp project:
Using .env files. (recommended)
Using shell. (useful for overrides)
1. Using .env (dotenv) Files
This is the recommended method for providing env vars to your Wasp project during development.
In the root of your Wasp project you can create two distinct files:
.env.server for env vars that will be provided to the server.
Variables are defined in these files in the form of NAME=VALUE, for example:
.env.server
DATABASE_URL=postgresql://localhost:5432
SOME_VAR_NAME=somevalue
.env.client for env vars that will be provided to the client.
Variables are defined in these files in the form of NAME=VALUE, for example:
.env.client
REACT_APP_SOME_VAR_NAME=somevalue
These files should not be committed to version control, and they are already ignored by default in the .gitignore file that comes with Wasp.
2. Using Shell
If you set environment variables in the shell where you run your Wasp commands (e.g., wasp start), Wasp will recognize them.
You can set environment variables in the .profile or a similar file, or by defining them at the start of a command:
SOME_VAR_NAME=SOMEVALUE wasp start
This is not specific to Wasp and is simply how environment variables can be set in the shell.
Defining environment variables in this way can be cumbersome even for a single project and even more challenging to manage if you have multiple Wasp projects. Therefore, we do not recommend this as a default method for providing environment variables to Wasp projects. However, it can be useful for occasionally overriding specific environment variables because environment variables set this way take precedence over those defined in .env files.
Defining Env Vars in Production
While in development, we had the option of using .env files which made it easy to define and manage env vars. However, in production, we need to provide env vars differently.
Client Env Vars
Client env vars are embedded into the client code during the build and shipping process, making them public and readable by anyone. Therefore, you should never store secrets in them (such as secret API keys).
You should provide them to the build command, for example:
REACT_APP_SOME_VAR_NAME=somevalue npm run build
How it works
What happens behind the scenes is that Wasp will replace all occurrences of import.meta.env.REACT_APP_SOME_VAR_NAME with the value you provided. This is done during the build process, so the value is embedded into the client code.
Read more about it in Vite's docs.
Server Env Vars
The way you provide env vars to your Wasp project in production depends on where you deploy it. For example, if you deploy your project to Fly, you can define them using the flyctl CLI tool:
flyctl secrets set SOME_VAR_NAME=somevalue
You can read a lot more details in the deployment section of the docs. We go into detail on how to define env vars for each deployment option. | https://wasp-lang.dev/docs/0.11.8/project/env-vars |
|
2 | null | 2024-04-23T15:15:20.334Z | https://wasp-lang.dev/docs/0.11.8/project/testing | https://wasp-lang.dev/docs/0.11.8 | http | Version: 0.11.8
## Testing
info
Wasp is in beta, so keep in mind there might be some kinks / bugs, and possibly some changes with testing support in the future. If you encounter any issues, reach out to us on [Discord](https://discord.gg/rzdnErX) and we will make sure to help you out!
## Testing Your React App[](#testing-your-react-app "Direct link to Testing Your React App")
Wasp enables you to quickly and easily write both unit tests and React component tests for your frontend code. Because Wasp uses [Vite](https://vitejs.dev/), we support testing web apps through [Vitest](https://vitest.dev/).
Included Libraries
### Writing Tests[](#writing-tests "Direct link to Writing Tests")
For Wasp to pick up your tests, they should be placed within the `src/client` directory and use an extension that matches [these glob patterns](https://vitest.dev/config#include). Some of the file names that Wasp will pick up as tests:
* `yourFile.test.ts`
* `YourComponent.spec.jsx`
Within test files, you can import your other source files as usual. For example, if you have a component `Counter.jsx`, you test it by creating a file in the same directory called `Counter.test.jsx` and import the component with `import Counter from './Counter'`.
### Running Tests[](#running-tests "Direct link to Running Tests")
Running `wasp test client` will start Vitest in watch mode and recompile your Wasp project when changes are made.
* If you want to see a realtime UI, pass `--ui` as an option.
* To run the tests just once, use `wasp test client run`.
All arguments after `wasp test client` are passed directly to the Vitest CLI, so check out [their documentation](https://vitest.dev/guide/cli.html) for all of the options.
Be Careful
You should not run `wasp test` while `wasp start` is running. Both will try to compile your project to `.wasp/out`.
### React Testing Helpers[](#react-testing-helpers "Direct link to React Testing Helpers")
Wasp provides several functions to help you write React tests:
* `renderInContext`: Takes a React component, wraps it inside a `QueryClientProvider` and `Router`, and renders it. This is the function you should use to render components in your React component tests.
```
import { renderInContext } from "@wasp/test";renderInContext(<MainPage />);
```
* `mockServer`: Sets up the mock server and returns an object containing the `mockQuery` and `mockApi` utilities. This should be called outside of any test case, in each file that wants to use those helpers.
```
import { mockServer } from "@wasp/test";const { mockQuery, mockApi } = mockServer();
```
* `mockQuery`: Takes a Wasp [query](https://wasp-lang.dev/docs/0.11.8/data-model/operations/queries) to mock and the JSON data it should return.
```
import getTasks from "@wasp/queries/getTasks";mockQuery(getTasks, []);
```
* Helpful when your component uses `useQuery`.
* Behind the scenes, Wasp uses [`msw`](https://npmjs.com/package/msw) to create a server request handle that responds with the specified data.
* Mock are cleared between each test.
* `mockApi`: Similar to `mockQuery`, but for [APIs](https://wasp-lang.dev/docs/0.11.8/advanced/apis). Instead of a Wasp query, it takes a route containing an HTTP method and a path.
```
import { HttpMethod } from "@wasp/types";mockApi({ method: HttpMethod.Get, path: "/foor/bar" }, { res: "hello" });
```
## Testing Your Server-Side Code[](#testing-your-server-side-code "Direct link to Testing Your Server-Side Code")
Wasp currently does not provide a way to test your server-side code, but we will be adding support soon. You can track the progress at [this GitHub issue](https://github.com/wasp-lang/wasp/issues/110) and express your interest by commenting.
## Examples[](#examples "Direct link to Examples")
You can see some tests in a Wasp project [here](https://github.com/wasp-lang/wasp/blob/release/waspc/examples/todoApp/src/client/pages/auth/helpers.test.ts).
### Client Unit Tests[](#client-unit-tests "Direct link to Client Unit Tests")
* JavaScript
* TypeScript
src/client/helpers.js
```
export function areThereAnyTasks(tasks) { return tasks.length === 0;}
```
src/client/helpers.test.js
```
import { test, expect } from "vitest";import { areThereAnyTasks } from "./helpers";test("areThereAnyTasks", () => { expect(areThereAnyTasks([])).toBe(false);});
```
### React Component Tests[](#react-component-tests "Direct link to React Component Tests")
* JavaScript
* TypeScript
src/client/Todo.jsx
```
import { useQuery } from "@wasp/queries";import getTasks from "@wasp/queries/getTasks";const Todo = (_props) => { const { data: tasks } = useQuery(getTasks); return ( <ul> {tasks && tasks.map((task) => ( <li key={task.id}> <input type="checkbox" value={task.isDone} /> {task.description} </li> ))} </ul> );};
```
src/client/Todo.test.jsx
```
import { test, expect } from "vitest";import { screen } from "@testing-library/react";import { mockServer, renderInContext } from "@wasp/test";import getTasks from "@wasp/queries/getTasks";import Todo from "./Todo";const { mockQuery } = mockServer();const mockTasks = [ { id: 1, description: "test todo 1", isDone: true, userId: 1, },];test("handles mock data", async () => { mockQuery(getTasks, mockTasks); renderInContext(<Todo />); await screen.findByText("test todo 1"); expect(screen.getByRole("checkbox")).toBeChecked(); screen.debug();});
```
### Testing With Mocked APIs[](#testing-with-mocked-apis "Direct link to Testing With Mocked APIs")
* JavaScript
* TypeScript
src/client/Todo.jsx
```
import api from "@wasp/api";const Todo = (_props) => { const [tasks, setTasks] = useState([]); useEffect(() => { api .get("/tasks") .then((res) => res.json()) .then((tasks) => setTasks(tasks)) .catch((err) => window.alert(err)); }); return ( <ul> {tasks && tasks.map((task) => ( <li key={task.id}> <input type="checkbox" value={task.isDone} /> {task.description} </li> ))} </ul> );};
```
src/client/Todo.test.jsx
```
import { test, expect } from "vitest";import { screen } from "@testing-library/react";import { mockServer, renderInContext } from "@wasp/test";import Todo from "./Todo";const { mockApi } = mockServer();const mockTasks = [ { id: 1, description: "test todo 1", isDone: true, userId: 1, },];test("handles mock data", async () => { mockApi("/tasks", { res: mockTasks }); renderInContext(<Todo />); await screen.findByText("test todo 1"); expect(screen.getByRole("checkbox")).toBeChecked(); screen.debug();});
``` | null | https://wasp-lang.dev/docs/0.11.8/project/testing | Wasp is in beta, so keep in mind there might be some kinks / bugs, and possibly some changes with testing support in the future. If you encounter any issues, reach out to us on Discord and we will make sure to help you out! | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecef3991d20ae-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:20 GMT | Tue, 23 Apr 2024 15:25:20 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=Zkwu%2Fh0HXrZxQi4boEEiaaYytih6D%2BbupHuE1hp4LpCH1gAorzuijy2o1jRZn5e09H6ZzXZMVWVY26MEzoyn9Gfv1PoVPz52TgP4O9oWZlxvqC7Q1ny0uE6bcfpUoCLz"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 71cf82a38fe87fe7b9fa7a7fc49613d9859d2f70 | null | 46F6:173D:DAE68:10ADB6:6627D088 | HIT | MISS | cache-iad-kiad7000030-IAD | S1713885320.275253,VS0,VE18 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/project/testing | og:url | Testing | Wasp | og:title | Wasp is in beta, so keep in mind there might be some kinks / bugs, and possibly some changes with testing support in the future. If you encounter any issues, reach out to us on Discord and we will make sure to help you out! | og:description | Testing | Wasp | null | Version: 0.11.8
Testing
info
Wasp is in beta, so keep in mind there might be some kinks / bugs, and possibly some changes with testing support in the future. If you encounter any issues, reach out to us on Discord and we will make sure to help you out!
Testing Your React App
Wasp enables you to quickly and easily write both unit tests and React component tests for your frontend code. Because Wasp uses Vite, we support testing web apps through Vitest.
Included Libraries
Writing Tests
For Wasp to pick up your tests, they should be placed within the src/client directory and use an extension that matches these glob patterns. Some of the file names that Wasp will pick up as tests:
yourFile.test.ts
YourComponent.spec.jsx
Within test files, you can import your other source files as usual. For example, if you have a component Counter.jsx, you test it by creating a file in the same directory called Counter.test.jsx and import the component with import Counter from './Counter'.
Running Tests
Running wasp test client will start Vitest in watch mode and recompile your Wasp project when changes are made.
If you want to see a realtime UI, pass --ui as an option.
To run the tests just once, use wasp test client run.
All arguments after wasp test client are passed directly to the Vitest CLI, so check out their documentation for all of the options.
Be Careful
You should not run wasp test while wasp start is running. Both will try to compile your project to .wasp/out.
React Testing Helpers
Wasp provides several functions to help you write React tests:
renderInContext: Takes a React component, wraps it inside a QueryClientProvider and Router, and renders it. This is the function you should use to render components in your React component tests.
import { renderInContext } from "@wasp/test";
renderInContext(<MainPage />);
mockServer: Sets up the mock server and returns an object containing the mockQuery and mockApi utilities. This should be called outside of any test case, in each file that wants to use those helpers.
import { mockServer } from "@wasp/test";
const { mockQuery, mockApi } = mockServer();
mockQuery: Takes a Wasp query to mock and the JSON data it should return.
import getTasks from "@wasp/queries/getTasks";
mockQuery(getTasks, []);
Helpful when your component uses useQuery.
Behind the scenes, Wasp uses msw to create a server request handle that responds with the specified data.
Mock are cleared between each test.
mockApi: Similar to mockQuery, but for APIs. Instead of a Wasp query, it takes a route containing an HTTP method and a path.
import { HttpMethod } from "@wasp/types";
mockApi({ method: HttpMethod.Get, path: "/foor/bar" }, { res: "hello" });
Testing Your Server-Side Code
Wasp currently does not provide a way to test your server-side code, but we will be adding support soon. You can track the progress at this GitHub issue and express your interest by commenting.
Examples
You can see some tests in a Wasp project here.
Client Unit Tests
JavaScript
TypeScript
src/client/helpers.js
export function areThereAnyTasks(tasks) {
return tasks.length === 0;
}
src/client/helpers.test.js
import { test, expect } from "vitest";
import { areThereAnyTasks } from "./helpers";
test("areThereAnyTasks", () => {
expect(areThereAnyTasks([])).toBe(false);
});
React Component Tests
JavaScript
TypeScript
src/client/Todo.jsx
import { useQuery } from "@wasp/queries";
import getTasks from "@wasp/queries/getTasks";
const Todo = (_props) => {
const { data: tasks } = useQuery(getTasks);
return (
<ul>
{tasks &&
tasks.map((task) => (
<li key={task.id}>
<input type="checkbox" value={task.isDone} />
{task.description}
</li>
))}
</ul>
);
};
src/client/Todo.test.jsx
import { test, expect } from "vitest";
import { screen } from "@testing-library/react";
import { mockServer, renderInContext } from "@wasp/test";
import getTasks from "@wasp/queries/getTasks";
import Todo from "./Todo";
const { mockQuery } = mockServer();
const mockTasks = [
{
id: 1,
description: "test todo 1",
isDone: true,
userId: 1,
},
];
test("handles mock data", async () => {
mockQuery(getTasks, mockTasks);
renderInContext(<Todo />);
await screen.findByText("test todo 1");
expect(screen.getByRole("checkbox")).toBeChecked();
screen.debug();
});
Testing With Mocked APIs
JavaScript
TypeScript
src/client/Todo.jsx
import api from "@wasp/api";
const Todo = (_props) => {
const [tasks, setTasks] = useState([]);
useEffect(() => {
api
.get("/tasks")
.then((res) => res.json())
.then((tasks) => setTasks(tasks))
.catch((err) => window.alert(err));
});
return (
<ul>
{tasks &&
tasks.map((task) => (
<li key={task.id}>
<input type="checkbox" value={task.isDone} />
{task.description}
</li>
))}
</ul>
);
};
src/client/Todo.test.jsx
import { test, expect } from "vitest";
import { screen } from "@testing-library/react";
import { mockServer, renderInContext } from "@wasp/test";
import Todo from "./Todo";
const { mockApi } = mockServer();
const mockTasks = [
{
id: 1,
description: "test todo 1",
isDone: true,
userId: 1,
},
];
test("handles mock data", async () => {
mockApi("/tasks", { res: mockTasks });
renderInContext(<Todo />);
await screen.findByText("test todo 1");
expect(screen.getByRole("checkbox")).toBeChecked();
screen.debug();
}); | https://wasp-lang.dev/docs/0.11.8/project/testing |
|
2 | null | 2024-04-23T15:15:20.171Z | https://wasp-lang.dev/docs/0.11.8/project/static-assets | https://wasp-lang.dev/docs/0.11.8 | http | ## Static Asset Handling
## Importing Asset as URL[](#importing-asset-as-url "Direct link to Importing Asset as URL")
Importing a static asset (e.g. an image) will return its URL. For example:
* JavaScript
* TypeScript
src/client/App.jsx
```
import imgUrl from './img.png'function App() { return <img src={imgUrl} alt="img" />}
```
For example, `imgUrl` will be `/img.png` during development, and become `/assets/img.2d8efhg.png` in the production build.
This is what you want to use most of the time, as it ensures that the asset file exists and is included in the bundle.
We are using Vite under the hood, read more about importing static assets in Vite's [docs](https://vitejs.dev/guide/assets.html#importing-asset-as-url).
## The `public` Directory[](#the-public-directory "Direct link to the-public-directory")
If you have assets that are:
* Never referenced in source code (e.g. robots.txt)
* Must retain the exact same file name (without hashing)
* ...or you simply don't want to have to import an asset first just to get its URL
Then you can place the asset in a special `public` directory in the `client` folder:
```
src└── client ├── public │ ├── favicon.ico │ └── robots.txt └── ...
```
Assets in this directory will be served at root path `/` during dev, and copied to the root of the dist directory as-is.
For example, if you have a file `favicon.ico` in the `public` directory, and your app is hosted at `https://myapp.com`, it will be made available at `https://myapp.com/favicon.ico`.
Usage in client code
Note that:
* You should always reference public assets using root absolute path - for example, `src/client/public/icon.png` should be referenced in source code as `/icon.png`.
* Assets in the `public` directory **cannot be imported** from . | null | https://wasp-lang.dev/docs/0.11.8/project/static-assets | Importing Asset as URL | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecef2b9390660-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:20 GMT | Tue, 23 Apr 2024 15:25:20 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=Q9RpL6ouw3W1Q11KgdTgho4aIG35Bw1TfpojG7dZezJmveRHF%2FrdTFm6fR993Bs0QwIxm6ZR4y6kDC8GYsj4gvR0%2Fu4tPrQWWNSmUgIiP8EoAG1y9gX%2BcTd3xi75wFy9"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | b06e4b723e0f5cb59e1265e4305851f4b867cd21 | null | 423E:32939:3DFEAD4:490533C:6627D087 | HIT | MISS | cache-iad-kiad7000148-IAD | S1713885320.135578,VS0,VE21 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/project/static-assets | og:url | Static Asset Handling | Wasp | og:title | Importing Asset as URL | og:description | Static Asset Handling | Wasp | null | Static Asset Handling
Importing Asset as URL
Importing a static asset (e.g. an image) will return its URL. For example:
JavaScript
TypeScript
src/client/App.jsx
import imgUrl from './img.png'
function App() {
return <img src={imgUrl} alt="img" />
}
For example, imgUrl will be /img.png during development, and become /assets/img.2d8efhg.png in the production build.
This is what you want to use most of the time, as it ensures that the asset file exists and is included in the bundle.
We are using Vite under the hood, read more about importing static assets in Vite's docs.
The public Directory
If you have assets that are:
Never referenced in source code (e.g. robots.txt)
Must retain the exact same file name (without hashing)
...or you simply don't want to have to import an asset first just to get its URL
Then you can place the asset in a special public directory in the client folder:
src
└── client
├── public
│ ├── favicon.ico
│ └── robots.txt
└── ...
Assets in this directory will be served at root path / during dev, and copied to the root of the dist directory as-is.
For example, if you have a file favicon.ico in the public directory, and your app is hosted at https://myapp.com, it will be made available at https://myapp.com/favicon.ico.
Usage in client code
Note that:
You should always reference public assets using root absolute path - for example, src/client/public/icon.png should be referenced in source code as /icon.png.
Assets in the public directory cannot be imported from . | https://wasp-lang.dev/docs/0.11.8/project/static-assets |
|
2 | null | 2024-04-23T15:15:21.239Z | https://wasp-lang.dev/docs/0.11.8/project/dependencies | https://wasp-lang.dev/docs/0.11.8 | http | In the current implementation of Wasp, if Wasp is already internally using a certain npm dependency with a certain version specified, you are not allowed to define that same npm dependency yourself while specifying _a different version_. If you do that, you will get an error message telling you which exact version you have to use for that dependency. This means Wasp _dictates exact versions of certain packages_, so for example you can't choose version of React you want to use.
We are currently working on a restructuring that will solve this and some other quirks that the current dependency system has: check [issue #734](https://github.com/wasp-lang/wasp/issues/734) to follow our progress. | null | https://wasp-lang.dev/docs/0.11.8/project/dependencies | Specifying npm dependencies in Wasp project is done via the dependencies field in the app declaration, in the following way: | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecef92f0907bb-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:21 GMT | Tue, 23 Apr 2024 15:25:21 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=pcYno8sFSya3ucVs3wjEDvXEeszFuaLILzzxwLyjWUysmZCWDnWcl7RSdOBbN9UNhTRIAMq6BUIeRuymoh6x8BBmOV%2BK0bq%2FOhX8jtC0JKTc9jFIGz6raSEFxXr1gb67"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 417efdf8647175555c77bc38ab2cf6319c5738fb | null | FA68:1736:590F51:6AE83F:6627D088 | HIT | MISS | cache-iad-kiad7000118-IAD | S1713885321.168921,VS0,VE43 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/project/dependencies | og:url | Dependencies | Wasp | og:title | Specifying npm dependencies in Wasp project is done via the dependencies field in the app declaration, in the following way: | og:description | Dependencies | Wasp | null | In the current implementation of Wasp, if Wasp is already internally using a certain npm dependency with a certain version specified, you are not allowed to define that same npm dependency yourself while specifying a different version. If you do that, you will get an error message telling you which exact version you have to use for that dependency. This means Wasp dictates exact versions of certain packages, so for example you can't choose version of React you want to use.
We are currently working on a restructuring that will solve this and some other quirks that the current dependency system has: check issue #734 to follow our progress. | https://wasp-lang.dev/docs/0.11.8/project/dependencies |
|
2 | null | 2024-04-23T15:15:23.096Z | https://wasp-lang.dev/docs/0.11.8/project/custom-vite-config | https://wasp-lang.dev/docs/0.11.8 | http | Version: 0.11.8
## Custom Vite Config
Wasp uses [Vite](https://vitejs.dev/) for serving the client during development and bundling it for production. If you want to customize the Vite config, you can do that by editing the `vite.config.ts` file in your `src/client` directory.
Wasp will use your config and **merge** it with the default Wasp's Vite config.
Vite config customization can be useful for things like:
* Adding custom Vite plugins.
* Customising the dev server.
* Customising the build process.
Be careful with making changes to the Vite config, as it can break the Wasp's client build process. Check out the default Vite config [here](https://github.com/wasp-lang/wasp/blob/main/waspc/data/Generator/templates/react-app/vite.config.ts) to see what you can change.
## Examples[](#examples "Direct link to Examples")
Below are some examples of how you can customize the Vite config.
### Changing the Dev Server Behaviour[](#changing-the-dev-server-behaviour "Direct link to Changing the Dev Server Behaviour")
If you want to stop Vite from opening the browser automatically when you run `wasp start`, you can do that by customizing the `open` option.
* JavaScript
* TypeScript
src/client/vite.config.js
```
export default { server: { open: false, },}
```
### Custom Dev Server Port[](#custom-dev-server-port "Direct link to Custom Dev Server Port")
You have access to all of the [Vite dev server options](https://vitejs.dev/config/server-options.html) in your custom Vite config. You can change the dev server port by setting the `port` option.
* JavaScript
* TypeScript
src/client/vite.config.js
```
export default { server: { port: 4000, },}
```
.env.server
```
WASP_WEB_CLIENT_URL=http://localhost:4000
```
Changing the dev server port
⚠️ Be careful when changing the dev server port, you'll need to update the `WASP_WEB_CLIENT_URL` env var in your `.env.server` file.
### Customising the Base Path[](#customising-the-base-path "Direct link to Customising the Base Path")
If you, for example, want to serve the client from a different path than `/`, you can do that by customizing the `base` option.
* JavaScript
* TypeScript
src/client/vite.config.js
```
export default { base: '/my-app/',}
``` | null | https://wasp-lang.dev/docs/0.11.8/project/custom-vite-config | Wasp uses Vite for serving the client during development and bundling it for production. If you want to customize the Vite config, you can do that by editing the vite.config.ts file in your src/client directory. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf05092b59a4-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:23 GMT | Tue, 23 Apr 2024 15:25:23 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=AAZggrk%2FnbvVvNXMvh4tyaH6%2B%2BSKOOe7xZKT7DApkt58Y3qn3GjYRNSLfioRV6NOmO4fuGusWVeIr7XLwk3M6L4qr0eP%2FzFH7S36mq87dBPfh%2FGLUAgYipTBS9DQbzv3"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 7804fa255beb684692deb0de66a56888eee445bc | null | 5558:2F9FB7:3A61CEA:457E78A:6627D08A | null | MISS | cache-iad-kiad7000121-IAD | S1713885323.065617,VS0,VE11 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/project/custom-vite-config | og:url | Custom Vite Config | Wasp | og:title | Wasp uses Vite for serving the client during development and bundling it for production. If you want to customize the Vite config, you can do that by editing the vite.config.ts file in your src/client directory. | og:description | Custom Vite Config | Wasp | null | Version: 0.11.8
Custom Vite Config
Wasp uses Vite for serving the client during development and bundling it for production. If you want to customize the Vite config, you can do that by editing the vite.config.ts file in your src/client directory.
Wasp will use your config and merge it with the default Wasp's Vite config.
Vite config customization can be useful for things like:
Adding custom Vite plugins.
Customising the dev server.
Customising the build process.
Be careful with making changes to the Vite config, as it can break the Wasp's client build process. Check out the default Vite config here to see what you can change.
Examples
Below are some examples of how you can customize the Vite config.
Changing the Dev Server Behaviour
If you want to stop Vite from opening the browser automatically when you run wasp start, you can do that by customizing the open option.
JavaScript
TypeScript
src/client/vite.config.js
export default {
server: {
open: false,
},
}
Custom Dev Server Port
You have access to all of the Vite dev server options in your custom Vite config. You can change the dev server port by setting the port option.
JavaScript
TypeScript
src/client/vite.config.js
export default {
server: {
port: 4000,
},
}
.env.server
WASP_WEB_CLIENT_URL=http://localhost:4000
Changing the dev server port
⚠️ Be careful when changing the dev server port, you'll need to update the WASP_WEB_CLIENT_URL env var in your .env.server file.
Customising the Base Path
If you, for example, want to serve the client from a different path than /, you can do that by customizing the base option.
JavaScript
TypeScript
src/client/vite.config.js
export default {
base: '/my-app/',
} | https://wasp-lang.dev/docs/0.11.8/project/custom-vite-config |
|
2 | null | 2024-04-23T15:15:23.150Z | https://wasp-lang.dev/docs/0.11.8/project/css-frameworks | https://wasp-lang.dev/docs/0.11.8 | http | ## CSS Frameworks
## Tailwind[](#tailwind "Direct link to Tailwind")
To enable support for Tailwind in your project, you need to add two config files — [`tailwind.config.cjs`](https://tailwindcss.com/docs/configuration#configuration-options) and `postcss.config.cjs` — to the root directory.
With these files present, Wasp installs the necessary dependencies and copies your configuration to the generated project. You can then use [Tailwind CSS directives](https://tailwindcss.com/docs/functions-and-directives#directives) in your CSS and Tailwind classes on your React components.
tree .
```
.├── main.wasp├── src│ ├── client│ │ ├── tsconfig.json│ │ ├── Main.css│ │ ├── MainPage.js│ │ └── waspLogo.png│ ├── server│ │ └── tsconfig.json│ └── shared│ └── tsconfig.json├── postcss.config.cjs└── tailwind.config.cjs
```
Tailwind not working?
If you can not use Tailwind after adding the required config files, make sure to restart `wasp start`. This is sometimes needed to ensure that Wasp picks up the changes and enables Tailwind integration.
### Enabling Tailwind Step-by-Step[](#enabling-tailwind-step-by-step "Direct link to Enabling Tailwind Step-by-Step")
caution
Make sure to use the `.cjs` extension for these config files, if you name them with a `.js` extension, Wasp will not detect them.
1. Add `./tailwind.config.cjs`.
./tailwind.config.cjs
```
/** @type {import('tailwindcss').Config} */module.exports = { content: [ "./src/**/*.{js,jsx,ts,tsx}" ], theme: { extend: {}, }, plugins: [],}
```
2. Add `./postcss.config.cjs`.
./postcss.config.cjs
```
module.exports = { plugins: { tailwindcss: {}, autoprefixer: {}, },}
```
3. Import Tailwind into your CSS file. For example, in a new project you might import Tailwind into `Main.css`.
./src/client/Main.css
```
@tailwind base;@tailwind components;@tailwind utilities;/* ... */
```
4. Start using Tailwind 🥳
./src/client/MainPage.jsx
```
// ...<h1 className="text-3xl font-bold underline"> Hello world!</h1>// ...
```
### Adding Tailwind Plugins[](#adding-tailwind-plugins "Direct link to Adding Tailwind Plugins")
To add Tailwind plugins, add it to [dependencies](https://wasp-lang.dev/docs/0.11.8/project/dependencies) in your `main.wasp` file and to the plugins list in your `tailwind.config.cjs` file:
./main.wasp
```
app todoApp { // ... dependencies: [ ("@tailwindcss/forms", "^0.5.3"), ("@tailwindcss/typography", "^0.5.7"), ], // ...}
```
./tailwind.config.cjs
```
/** @type {import('tailwindcss').Config} */module.exports = { // ... plugins: [ require('@tailwindcss/forms'), require('@tailwindcss/typography'), ], // ...}
``` | null | https://wasp-lang.dev/docs/0.11.8/project/css-frameworks | Tailwind | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf055ef720d1-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:23 GMT | Tue, 23 Apr 2024 15:25:23 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=iBptb8BzDrTg4k5zdeh%2BpHY3Ra028rYw3THaTT9iROXaJx9UoIjcKFEvj62O0hlK6xXHpTMu6yKvMGK2MfRqcaTCNlo%2Bohp%2FjfvudOyGj%2FXTbzJqK5Ye%2FgQv%2FmiNtUIu"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | ec2d7358fef25870031bd3e7093c836c2787c6d8 | null | 538C:3E1868:3B38A7A:463EB76:6627D08B | HIT | MISS | cache-iad-kiad7000151-IAD | S1713885323.122865,VS0,VE11 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/project/css-frameworks | og:url | CSS Frameworks | Wasp | og:title | Tailwind | og:description | CSS Frameworks | Wasp | null | CSS Frameworks
Tailwind
To enable support for Tailwind in your project, you need to add two config files — tailwind.config.cjs and postcss.config.cjs — to the root directory.
With these files present, Wasp installs the necessary dependencies and copies your configuration to the generated project. You can then use Tailwind CSS directives in your CSS and Tailwind classes on your React components.
tree .
.
├── main.wasp
├── src
│ ├── client
│ │ ├── tsconfig.json
│ │ ├── Main.css
│ │ ├── MainPage.js
│ │ └── waspLogo.png
│ ├── server
│ │ └── tsconfig.json
│ └── shared
│ └── tsconfig.json
├── postcss.config.cjs
└── tailwind.config.cjs
Tailwind not working?
If you can not use Tailwind after adding the required config files, make sure to restart wasp start. This is sometimes needed to ensure that Wasp picks up the changes and enables Tailwind integration.
Enabling Tailwind Step-by-Step
caution
Make sure to use the .cjs extension for these config files, if you name them with a .js extension, Wasp will not detect them.
Add ./tailwind.config.cjs.
./tailwind.config.cjs
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [ "./src/**/*.{js,jsx,ts,tsx}" ],
theme: {
extend: {},
},
plugins: [],
}
Add ./postcss.config.cjs.
./postcss.config.cjs
module.exports = {
plugins: {
tailwindcss: {},
autoprefixer: {},
},
}
Import Tailwind into your CSS file. For example, in a new project you might import Tailwind into Main.css.
./src/client/Main.css
@tailwind base;
@tailwind components;
@tailwind utilities;
/* ... */
Start using Tailwind 🥳
./src/client/MainPage.jsx
// ...
<h1 className="text-3xl font-bold underline">
Hello world!
</h1>
// ...
Adding Tailwind Plugins
To add Tailwind plugins, add it to dependencies in your main.wasp file and to the plugins list in your tailwind.config.cjs file:
./main.wasp
app todoApp {
// ...
dependencies: [
("@tailwindcss/forms", "^0.5.3"),
("@tailwindcss/typography", "^0.5.7"),
],
// ...
}
./tailwind.config.cjs
/** @type {import('tailwindcss').Config} */
module.exports = {
// ...
plugins: [
require('@tailwindcss/forms'),
require('@tailwindcss/typography'),
],
// ...
} | https://wasp-lang.dev/docs/0.11.8/project/css-frameworks |
|
2 | null | 2024-04-23T15:15:23.681Z | https://wasp-lang.dev/docs/0.11.8/advanced/deployment/overview | https://wasp-lang.dev/docs/0.11.8 | http | ## Overview
Wasp apps are full-stack apps that consist of:
* A Node.js server.
* A static client.
* A PostgreSQL database.
You can deploy each part **anywhere** where you can usually deploy Node.js apps or static apps. For example, you can deploy your client on [Netlify](https://www.netlify.com/), the server on [Fly.io](https://fly.io/), and the database on [Neon](https://neon.tech/).
To make deploying as smooth as possible, Wasp also offers a single-command deployment through the **Wasp CLI**. Read more about deploying through the CLI [here](https://wasp-lang.dev/docs/0.11.8/advanced/deployment/cli).
Click on each deployment method for more details.
Regardless of how you choose to deploy your app (i.e., manually or using the Wasp CLI), you'll need to know about some common patterns covered below.
## Customizing the Dockerfile[](#customizing-the-dockerfile "Direct link to Customizing the Dockerfile")
By default, Wasp generates a multi-stage Dockerfile. This file is used to build and run a Docker image with the Wasp-generated server code. It also runs any pending migrations.
You can **add extra steps to this multi-stage `Dockerfile`** by creating your own `Dockerfile` in the project's root directory. If Wasp finds a Dockerfile in the project's root, it appends its contents at the _bottom_ of the default multi-stage Dockerfile.
Since the last definition in a Dockerfile wins, you can override or continue from any existing build stages. You can also choose not to use any of our build stages and have your own custom Dockerfile used as-is.
A few things to keep in mind:
* If you override an intermediate build stage, no later build stages will be used unless you reproduce them below.
* The generated Dockerfile's content is dynamic and depends on which features your app uses. The content can also change in future releases, so please verify it from time to time.
* Make sure to supply `ENTRYPOINT` in your final build stage. Your changes won't have any effect if you don't.
Read more in the official Docker docs on [multi-stage builds](https://docs.docker.com/build/building/multi-stage/).
To see what your project's (potentially combined) Dockerfile will look like, run:
Join our [Discord](https://discord.gg/rzdnErX) if you have any questions, or if you need more customization than this hook provides. | null | https://wasp-lang.dev/docs/0.11.8/advanced/deployment/overview | Wasp apps are full-stack apps that consist of: | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf08ad6f576a-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:23 GMT | Tue, 23 Apr 2024 15:25:23 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=vCJXDJByP%2BnomNXlQgEW6y1y19aE8sgqtpC3dQkBOrjwYHG8FiVbUwItXOsKtiUujUAti2aQ5r3uNiwFC5VVBfu9ePlEhVX3FtBkG%2FAbBN2DKeVtCcsBKhL38t2lwjvc"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | a06ab27868d16aedc8caa6712ee81720414713f7 | null | 0C8A:318A9F:3B06E07:460CA59:6627D08B | HIT | MISS | cache-iad-kiad7000125-IAD | S1713885324.652343,VS0,VE15 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/advanced/deployment/overview | og:url | Overview | Wasp | og:title | Wasp apps are full-stack apps that consist of: | og:description | Overview | Wasp | null | Overview
Wasp apps are full-stack apps that consist of:
A Node.js server.
A static client.
A PostgreSQL database.
You can deploy each part anywhere where you can usually deploy Node.js apps or static apps. For example, you can deploy your client on Netlify, the server on Fly.io, and the database on Neon.
To make deploying as smooth as possible, Wasp also offers a single-command deployment through the Wasp CLI. Read more about deploying through the CLI here.
Click on each deployment method for more details.
Regardless of how you choose to deploy your app (i.e., manually or using the Wasp CLI), you'll need to know about some common patterns covered below.
Customizing the Dockerfile
By default, Wasp generates a multi-stage Dockerfile. This file is used to build and run a Docker image with the Wasp-generated server code. It also runs any pending migrations.
You can add extra steps to this multi-stage Dockerfile by creating your own Dockerfile in the project's root directory. If Wasp finds a Dockerfile in the project's root, it appends its contents at the bottom of the default multi-stage Dockerfile.
Since the last definition in a Dockerfile wins, you can override or continue from any existing build stages. You can also choose not to use any of our build stages and have your own custom Dockerfile used as-is.
A few things to keep in mind:
If you override an intermediate build stage, no later build stages will be used unless you reproduce them below.
The generated Dockerfile's content is dynamic and depends on which features your app uses. The content can also change in future releases, so please verify it from time to time.
Make sure to supply ENTRYPOINT in your final build stage. Your changes won't have any effect if you don't.
Read more in the official Docker docs on multi-stage builds.
To see what your project's (potentially combined) Dockerfile will look like, run:
Join our Discord if you have any questions, or if you need more customization than this hook provides. | https://wasp-lang.dev/docs/0.11.8/advanced/deployment/overview |
|
2 | null | 2024-04-23T15:15:25.148Z | https://wasp-lang.dev/docs/0.11.8/advanced/deployment/manually | https://wasp-lang.dev/docs/0.11.8 | http | ## Deploying Manually
We'll cover how to deploy your Wasp app manually to a variety of providers:
* [Fly.io](#flyio)
* [Netlify](#netlify)
* [Railway](#railway)
* [Heroku](#heroku)
## Deploying a Wasp App[](#deploying-a-wasp-app "Direct link to Deploying a Wasp App")
Deploying a Wasp app comes down to the following:
1. Generating deployable code.
2. Deploying the API server (backend).
3. Deploying the web client (frontend).
4. Deploying a PostgreSQL database and keeping it running.
Let's go through each of these steps.
### 1\. Generating Deployable Code[](#1-generating-deployable-code "Direct link to 1. Generating Deployable Code")
Running the command `wasp build` generates deployable code for the whole app in the `.wasp/build/` directory.
PostgreSQL in production
You won't be able to build the app if you are using SQLite as a database (which is the default database). You'll have to [switch to PostgreSQL](https://wasp-lang.dev/docs/0.11.8/data-model/backends#migrating-from-sqlite-to-postgresql) before deploying to production.
### 2\. Deploying the API Server (backend)[](#2-deploying-the-api-server-backend "Direct link to 2. Deploying the API Server (backend)")
There's a Dockerfile that defines an image for building the server in the `.wasp/build` directory.
To run the server in production, deploy this Docker image to a hosting provider and ensure it has access to correct environment variables (this varies depending on the provider). All necessary environment variables are listed in the next section.
#### Environment Variables[](#environment-variables "Direct link to Environment Variables")
Here are the environment variables your server requires to run:
* `PORT`
The server's HTTP port number. This is where the server listens for requests (e.g., `3001`).
* `DATABASE_URL`
The URL of the Postgres database you want your app to use (e.g., `postgresql://mydbuser:mypass@localhost:5432/nameofmydb`).
* `WASP_WEB_CLIENT_URL`
The URL where you plan to deploy your frontend app is running (e.g., `https://<app-name>.netlify.app`). The server needs to know about it to properly configure Same-Origin Policy (CORS) headers.
* `JWT_SECRET`
You only need this environment variable if you're using Wasp's `auth` features. Set it to a random string at least 32 characters long (you can use an [online generator](https://djecrety.ir/)).
Using an external auth method?
If your app is using an external authentication method(s) supported by Wasp (such as [Google](https://wasp-lang.dev/docs/0.11.8/auth/social-auth/google#4-adding-environment-variables) or [GitHub](https://wasp-lang.dev/docs/0.11.8/auth/social-auth/github#4-adding-environment-variables)), make sure to set the necessary environment variables.
### 3\. Deploying the Web Client (frontend)[](#3-deploying-the-web-client-frontend "Direct link to 3. Deploying the Web Client (frontend)")
To build the web app, position yourself in `.wasp/build/web-app` directory:
Run
```
npm install && REACT_APP_API_URL=<url_to_wasp_backend> npm run build
```
where `<url_to_wasp_backend>` is the URL of the Wasp server that you previously deployed.
The command above will build the web client and put it in the `build/` directory in the `web-app` directory.
Since the app's frontend is just a bunch of static files, you can deploy it to any static hosting provider.
### 4\. Deploying the Database[](#4-deploying-the-database "Direct link to 4. Deploying the Database")
Any PostgreSQL database will do, as long as you set the `DATABASE_URL` env var correctly and ensure that the database is accessible from the server.
## Different Providers[](#different-providers "Direct link to Different Providers")
We'll cover a few different deployment providers below:
* Fly.io (server and database)
* Netlify (client)
* Railway (server, client and database)
* Heroku (server and database)
## Fly.io[](#flyio "Direct link to Fly.io")
We automated this process for you
If you want to do all of the work below with one command, you can use the [Wasp CLI](https://wasp-lang.dev/docs/0.11.8/advanced/deployment/cli#flyio).
Wasp CLI deploys the server, deploys the client, and sets up a database. It also gives you a way to redeploy (update) your app with a single command.
Fly.io offers a variety of free services that are perfect for deploying your first Wasp app! You will need a Fly.io account and the [`flyctl` CLI](https://fly.io/docs/hands-on/install-flyctl/).
note
Fly.io offers support for both locally built Docker containers and remotely built ones. However, for simplicity and reproducibility, we will default to the use of a remote Fly.io builder.
Additionally, `fly` is a symlink for `flyctl` on most systems and they can be used interchangeably.
Make sure you are logged in with `flyctl` CLI. You can check if you are logged in with `flyctl auth whoami`, and if you are not, you can log in with `flyctl auth login`.
### Set Up a Fly.io App[](#set-up-a-flyio-app "Direct link to Set Up a Fly.io App")
info
You need to do this only once per Wasp app.
Unless you already have a Fly.io app that you want to deploy to, let's create a new Fly.io app.
After you have [built the app](#1-generating-deployable-code), position yourself in `.wasp/build/` directory:
Next, run the launch command to set up a new app and create a `fly.toml` file:
```
flyctl launch --remote-only
```
This will ask you a series of questions, such as asking you to choose a region and whether you'd like a database.
* Say **yes** to **Would you like to set up a Postgresql database now?** and select **Development**. Fly.io will set a `DATABASE_URL` for you.
* Say **no** to **Would you like to deploy now?** (and to any additional questions).
We still need to set up several environment variables.
What if the database setup fails?
If your attempts to initiate a new app fail for whatever reason, then you should run `flyctl apps destroy <app-name>` before trying again. Fly does not allow you to create multiple apps with the same name.
What does it look like when your DB is deployed correctly?
Next, let's copy the `fly.toml` file up to our Wasp project dir for safekeeping.
Next, let's add a few more environment variables:
```
flyctl secrets set PORT=8080flyctl secrets set JWT_SECRET=<random_string_at_least_32_characters_long>flyctl secrets set WASP_WEB_CLIENT_URL=<url_of_where_frontend_will_be_deployed>
```
note
If you do not know what your frontend URL is yet, don't worry. You can set `WASP_WEB_CLIENT_URL` after you deploy your frontend.
Using an external auth method?
If your app is using an external authentication method(s) supported by Wasp (such as [Google](https://wasp-lang.dev/docs/0.11.8/auth/social-auth/google#4-adding-environment-variables) or [GitHub](https://wasp-lang.dev/docs/0.11.8/auth/social-auth/github#4-adding-environment-variables)), make sure to set the necessary environment variables.
If you want to make sure you've added your secrets correctly, run `flyctl secrets list` in the terminal. Note that you will see hashed versions of your secrets to protect your sensitive data.
### Deploy to a Fly.io App[](#deploy-to-a-flyio-app "Direct link to Deploy to a Fly.io App")
While still in the `.wasp/build/` directory, run:
```
flyctl deploy --remote-only --config ../../fly.toml
```
This will build and deploy the backend of your Wasp app on Fly.io to `https://<app-name>.fly.dev` 🤘🎸
Now, if you haven't, you can deploy your frontend and add the client url by running `flyctl secrets set WASP_WEB_CLIENT_URL=<url_of_deployed_frontend>`. We suggest using [Netlify](#netlify) for your frontend, but you can use any static hosting provider.
Additionally, some useful `flyctl` commands:
```
flyctl logsflyctl secrets listflyctl ssh console
```
### Redeploying After Wasp Builds[](#redeploying-after-wasp-builds "Direct link to Redeploying After Wasp Builds")
When you rebuild your Wasp app (with `wasp build`), it will remove your `.wasp/build/` directory. In there, you may have a `fly.toml` from any prior Fly.io deployments.
While we will improve this process in the future, in the meantime, you have a few options:
1. Copy the `fly.toml` file to a versioned directory, like your Wasp project dir.
From there, you can reference it in `flyctl deploy --config <path>` commands, like above.
2. Backup the `fly.toml` file somewhere before running `wasp build`, and copy it into .wasp/build/ after.
When the `fly.toml` file exists in .wasp/build/ dir, you do not need to specify the `--config <path>`.
3. Run `flyctl config save -a <app-name>` to regenerate the `fly.toml` file from the remote state stored in Fly.io.
## Netlify[](#netlify "Direct link to Netlify")
Netlify is a static hosting solution that is free for many use cases. You will need a Netlify account and [Netlify CLI](https://docs.netlify.com/cli/get-started/) installed to follow these instructions.
Make sure you are logged in with Netlify CLI. You can check if you are logged in with `netlify status`, and if you are not, you can log in with `netlify login`.
First, make sure you have [built the Wasp app](#1-generating-deployable-code). We'll build the client web app next.
To build the web app, position yourself in `.wasp/build/web-app` directory:
Run
```
npm install && REACT_APP_API_URL=<url_to_wasp_backend> npm run build
```
where `<url_to_wasp_backend>` is the URL of the Wasp server that you previously deployed.
We can now deploy the client with:
Carefully follow the instructions i.e. do you want to create a new app or use an existing one, the team under which your app will reside etc.
The final step is to run:
That is it! Your client should be live at `https://<app-name>.netlify.app` ✨
note
Make sure you set this URL as the `WASP_WEB_CLIENT_URL` environment variable in your server hosting environment (e.g., Fly.io or Heroku).
## Railway[](#railway "Direct link to Railway")
Railway is a simple and great way to host your server and database. It's also possible to deploy your entire app: database, server, and client. You can use the platform for free for a limited time, or if you meet certain eligibility requirements. See their [plans page](https://docs.railway.app/reference/plans) for more info.
### Prerequisites[](#prerequisites "Direct link to Prerequisites")
To get started, follow these steps:
1. Make sure your Wasp app is built by running `wasp build` in the project dir.
2. Create a [Railway](https://railway.app/) account
Free Tier
Sign up with your GitHub account to be eligible for the free tier
3. Install the [Railway CLI](https://docs.railway.app/develop/cli#installation)
4. Run `railway login` and a browser tab will open to authenticate you.
### Create New Project[](#create-new-project "Direct link to Create New Project")
Let's create our Railway project:
1. Go to your [Railway dashboard](https://railway.app/dashboard), click on **New Project**, and select `Provision PostgreSQL` from the dropdown menu.
2. Once it initializes, right-click on the **New** button in the top right corner and select **Empty Service**.
3. Once it initializes, click on it, go to **Settings > General** and change the name to `server`
4. Go ahead and create another empty service and name it `client`
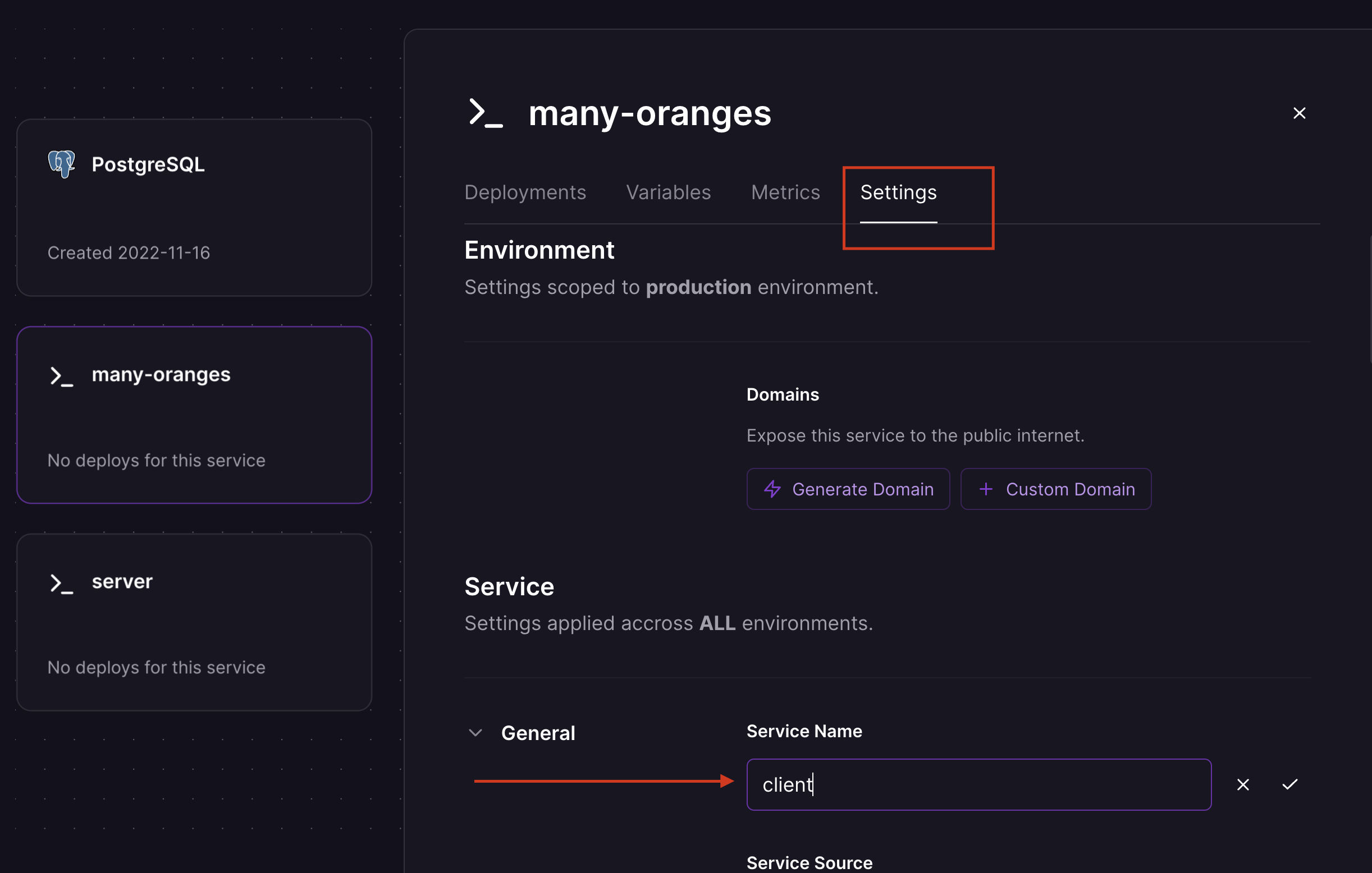
### Deploy Your App to Railway[](#deploy-your-app-to-railway "Direct link to Deploy Your App to Railway")
#### Setup Domains[](#setup-domains "Direct link to Setup Domains")
We'll need the domains for both the `server` and `client` services:
1. Go to the `server` instance's `Settings` tab, and click `Generate Domain`.
2. Do the same under the `client`'s `Settings`.
Copy the domains as we will need them later.
#### Deploying the Server[](#deploying-the-server "Direct link to Deploying the Server")
Let's deploy our server first:
1. Move into your app's `.wasp/build/` directory:
2. Link your app build to your newly created Railway project:
3. Go into the Railway dashboard and set up the required env variables:
Open the `Settings` and go to the `Variables` tab:
* click **Variable reference** and select `DATABASE_URL` (it will populate it with the correct value)
* add `WASP_WEB_CLIENT_URL` - enter the the `client` domain (e.g. `https://client-production-XXXX.up.railway.app`)
* add `JWT_SECRET` - enter a random string at least 32 characters long (use an [online generator](https://djecrety.ir/))
Using an external auth method?
If your app is using an external authentication method(s) supported by Wasp (such as [Google](https://wasp-lang.dev/docs/0.11.8/auth/social-auth/google#4-adding-environment-variables) or [GitHub](https://wasp-lang.dev/docs/0.11.8/auth/social-auth/github#4-adding-environment-variables)), make sure to set the necessary environment variables.
4. Push and deploy the project:
Select `server` when prompted with `Select Service`.
Railway will now locate the Dockerfile and deploy your server 👍
#### Deploying the Client[](#deploying-the-client "Direct link to Deploying the Client")
1. Next, change into your app's frontend build directory `.wasp/build/web-app`:
2. Create the production build, using the `server` domain as the `REACT_APP_API_URL`:
```
npm install && REACT_APP_API_URL=<url_to_wasp_backend> npm run build
```
3. Next, we want to link this specific frontend directory to our project as well:
4. We need to configure Railway's static hosting for our client.
Setting Up Static Hosting
Copy the `build` folder within the `web-app` directory to `dist`:
We'll need to create the following files:
* `Dockerfile` with:
Dockerfile
```
FROM pierrezemb/gostaticCMD [ "-fallback", "index.html" ]COPY ./dist/ /srv/http/
```
* `.dockerignore` with:
You'll need to repeat these steps **each time** you run `wasp build` as it will remove the `.wasp/build/web-app` directory.
Here's a useful shell script to do the process
If you want to automate the process, save the following as `deploy_client.sh` in the root of your project:
deploy\_client.sh
```
#!/usr/bin/env bashif [ -z "$REACT_APP_API_URL" ]then echo "REACT_APP_API_URL is not set" exit 1fiwasp buildcd .wasp/build/web-appnpm install && REACT_APP_API_URL=$REACT_APP_API_URL npm run buildcp -r build distdockerfile_contents=$(cat <<EOFFROM pierrezemb/gostaticCMD [ "-fallback", "index.html" ]COPY ./dist/ /srv/http/EOF)dockerignore_contents=$(cat <<EOFnode_modules/EOF)echo "$dockerfile_contents" > Dockerfileecho "$dockerignore_contents" > .dockerignorerailway up
```
Make it executable with:
```
chmod +x deploy_client.sh
```
You can run it with:
```
REACT_APP_API_URL=<url_to_wasp_backend> ./deploy_client.sh
```
5. Set the `PORT` environment variable to `8043` under the `Variables` tab.
6. Deploy the client and select `client` when prompted with `Select Service`:
#### Conclusion[](#conclusion "Direct link to Conclusion")
And now your Wasp should be deployed! 🐝 🚂 🚀
Back in your [Railway dashboard](https://railway.app/dashboard), click on your project and you should see your newly deployed services: Postgres, Server, and Client.
### Updates & Redeploying[](#updates--redeploying "Direct link to Updates & Redeploying")
When you make updates and need to redeploy:
* run `wasp build` to rebuild your app
* run `railway up` in the `.wasp/build` directory (server)
* repeat all the steps in the `.wasp/build/web-app` directory (client)
## Heroku[](#heroku "Direct link to Heroku")
note
Heroku used to offer free apps under certain limits. However, as of November 28, 2022, they ended support for their free tier. [https://blog.heroku.com/next-chapter](https://blog.heroku.com/next-chapter)
As such, we recommend using an alternative provider like [Fly.io](#flyio) for your first apps.
You will need Heroku account, `heroku` [CLI](https://devcenter.heroku.com/articles/heroku-cli) and `docker` CLI installed to follow these instructions.
Make sure you are logged in with `heroku` CLI. You can check if you are logged in with `heroku whoami`, and if you are not, you can log in with `heroku login`.
### Set Up a Heroku App[](#set-up-a-heroku-app "Direct link to Set Up a Heroku App")
info
You need to do this only once per Wasp app.
Unless you want to deploy to an existing Heroku app, let's create a new Heroku app:
Unless you have an external Postgres database that you want to use, let's create a new database on Heroku and attach it to our app:
```
heroku addons:create --app <app-name> heroku-postgresql:mini
```
caution
Heroku does not offer a free plan anymore and `mini` is their cheapest database instance - it costs $5/mo.
Heroku will also set `DATABASE_URL` env var for us at this point. If you are using an external database, you will have to set it up yourself.
The `PORT` env var will also be provided by Heroku, so the only two left to set are the `JWT_SECRET` and `WASP_WEB_CLIENT_URL` env vars:
```
heroku config:set --app <app-name> JWT_SECRET=<random_string_at_least_32_characters_long>heroku config:set --app <app-name> WASP_WEB_CLIENT_URL=<url_of_where_frontend_will_be_deployed>
```
note
If you do not know what your frontend URL is yet, don't worry. You can set `WASP_WEB_CLIENT_URL` after you deploy your frontend.
### Deploy to a Heroku App[](#deploy-to-a-heroku-app "Direct link to Deploy to a Heroku App")
After you have [built the app](#1-generating-deployable-code), position yourself in `.wasp/build/` directory:
assuming you were at the root of your Wasp project at that moment.
Log in to Heroku Container Registry:
Build the docker image and push it to Heroku:
```
heroku container:push --app <app-name> web
```
App is still not deployed at this point. This step might take some time, especially the very first time, since there are no cached docker layers.
Note for Apple Silicon Users
Apple Silicon users need to build a non-Arm image, so the above step will not work at this time. Instead of `heroku container:push`, users instead should:
```
docker buildx build --platform linux/amd64 -t <app-name> .docker tag <app-name> registry.heroku.com/<app-name>/webdocker push registry.heroku.com/<app-name>/web
```
You are now ready to proceed to the next step.
Deploy the pushed image and restart the app:
```
heroku container:release --app <app-name> web
```
This is it, the backend is deployed at `https://<app-name>-XXXX.herokuapp.com` 🎉
Find out the exact app URL with:
```
heroku info --app <app-name>
```
Additionally, you can check out the logs with:
```
heroku logs --tail --app <app-name>
```
Using `pg-boss` with Heroku
If you wish to deploy an app leveraging [Jobs](https://wasp-lang.dev/docs/0.11.8/advanced/jobs) that use `pg-boss` as the executor to Heroku, you need to set an additional environment variable called `PG_BOSS_NEW_OPTIONS` to `{"connectionString":"<REGULAR_HEROKU_DATABASE_URL>","ssl":{"rejectUnauthorized":false}}`. This is because pg-boss uses the `pg` extension, which does not seem to connect to Heroku over SSL by default, which Heroku requires. Additionally, Heroku uses a self-signed cert, so we must handle that as well.
Read more: [https://devcenter.heroku.com/articles/connecting-heroku-postgres#connecting-in-node-js](https://devcenter.heroku.com/articles/connecting-heroku-postgres#connecting-in-node-js) | null | https://wasp-lang.dev/docs/0.11.8/advanced/deployment/manually | We'll cover how to deploy your Wasp app manually to a variety of providers: | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf11b92120d6-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:25 GMT | Tue, 23 Apr 2024 15:25:25 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=3BCxcKTe%2BrwyzMZ%2BxFR%2Ff3nRtXt7Udza41fE%2FvXYvmtKbzmQxn6%2BGy7E9mF%2FhiEjjP9bpaiKw91fV%2BITVsPsxsNYUhfIV3toroVapI1Ih4QjcmSiCIradXvIKETCT%2B%2Fi"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 9220642fac23bb76f531e2c6b27cc1cce4c1e09d | null | A3CC:1FE0FF:3C0BB16:471180E:6627D08C | HIT | MISS | cache-iad-kiad7000144-IAD | S1713885325.094038,VS0,VE19 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/advanced/deployment/manually | og:url | Deploying Manually | Wasp | og:title | We'll cover how to deploy your Wasp app manually to a variety of providers: | og:description | Deploying Manually | Wasp | null | Deploying Manually
We'll cover how to deploy your Wasp app manually to a variety of providers:
Fly.io
Netlify
Railway
Heroku
Deploying a Wasp App
Deploying a Wasp app comes down to the following:
Generating deployable code.
Deploying the API server (backend).
Deploying the web client (frontend).
Deploying a PostgreSQL database and keeping it running.
Let's go through each of these steps.
1. Generating Deployable Code
Running the command wasp build generates deployable code for the whole app in the .wasp/build/ directory.
PostgreSQL in production
You won't be able to build the app if you are using SQLite as a database (which is the default database). You'll have to switch to PostgreSQL before deploying to production.
2. Deploying the API Server (backend)
There's a Dockerfile that defines an image for building the server in the .wasp/build directory.
To run the server in production, deploy this Docker image to a hosting provider and ensure it has access to correct environment variables (this varies depending on the provider). All necessary environment variables are listed in the next section.
Environment Variables
Here are the environment variables your server requires to run:
PORT
The server's HTTP port number. This is where the server listens for requests (e.g., 3001).
DATABASE_URL
The URL of the Postgres database you want your app to use (e.g., postgresql://mydbuser:mypass@localhost:5432/nameofmydb).
WASP_WEB_CLIENT_URL
The URL where you plan to deploy your frontend app is running (e.g., https://<app-name>.netlify.app). The server needs to know about it to properly configure Same-Origin Policy (CORS) headers.
JWT_SECRET
You only need this environment variable if you're using Wasp's auth features. Set it to a random string at least 32 characters long (you can use an online generator).
Using an external auth method?
If your app is using an external authentication method(s) supported by Wasp (such as Google or GitHub), make sure to set the necessary environment variables.
3. Deploying the Web Client (frontend)
To build the web app, position yourself in .wasp/build/web-app directory:
Run
npm install && REACT_APP_API_URL=<url_to_wasp_backend> npm run build
where <url_to_wasp_backend> is the URL of the Wasp server that you previously deployed.
The command above will build the web client and put it in the build/ directory in the web-app directory.
Since the app's frontend is just a bunch of static files, you can deploy it to any static hosting provider.
4. Deploying the Database
Any PostgreSQL database will do, as long as you set the DATABASE_URL env var correctly and ensure that the database is accessible from the server.
Different Providers
We'll cover a few different deployment providers below:
Fly.io (server and database)
Netlify (client)
Railway (server, client and database)
Heroku (server and database)
Fly.io
We automated this process for you
If you want to do all of the work below with one command, you can use the Wasp CLI.
Wasp CLI deploys the server, deploys the client, and sets up a database. It also gives you a way to redeploy (update) your app with a single command.
Fly.io offers a variety of free services that are perfect for deploying your first Wasp app! You will need a Fly.io account and the flyctl CLI.
note
Fly.io offers support for both locally built Docker containers and remotely built ones. However, for simplicity and reproducibility, we will default to the use of a remote Fly.io builder.
Additionally, fly is a symlink for flyctl on most systems and they can be used interchangeably.
Make sure you are logged in with flyctl CLI. You can check if you are logged in with flyctl auth whoami, and if you are not, you can log in with flyctl auth login.
Set Up a Fly.io App
info
You need to do this only once per Wasp app.
Unless you already have a Fly.io app that you want to deploy to, let's create a new Fly.io app.
After you have built the app, position yourself in .wasp/build/ directory:
Next, run the launch command to set up a new app and create a fly.toml file:
flyctl launch --remote-only
This will ask you a series of questions, such as asking you to choose a region and whether you'd like a database.
Say yes to Would you like to set up a Postgresql database now? and select Development. Fly.io will set a DATABASE_URL for you.
Say no to Would you like to deploy now? (and to any additional questions).
We still need to set up several environment variables.
What if the database setup fails?
If your attempts to initiate a new app fail for whatever reason, then you should run flyctl apps destroy <app-name> before trying again. Fly does not allow you to create multiple apps with the same name.
What does it look like when your DB is deployed correctly?
Next, let's copy the fly.toml file up to our Wasp project dir for safekeeping.
Next, let's add a few more environment variables:
flyctl secrets set PORT=8080
flyctl secrets set JWT_SECRET=<random_string_at_least_32_characters_long>
flyctl secrets set WASP_WEB_CLIENT_URL=<url_of_where_frontend_will_be_deployed>
note
If you do not know what your frontend URL is yet, don't worry. You can set WASP_WEB_CLIENT_URL after you deploy your frontend.
Using an external auth method?
If your app is using an external authentication method(s) supported by Wasp (such as Google or GitHub), make sure to set the necessary environment variables.
If you want to make sure you've added your secrets correctly, run flyctl secrets list in the terminal. Note that you will see hashed versions of your secrets to protect your sensitive data.
Deploy to a Fly.io App
While still in the .wasp/build/ directory, run:
flyctl deploy --remote-only --config ../../fly.toml
This will build and deploy the backend of your Wasp app on Fly.io to https://<app-name>.fly.dev 🤘🎸
Now, if you haven't, you can deploy your frontend and add the client url by running flyctl secrets set WASP_WEB_CLIENT_URL=<url_of_deployed_frontend>. We suggest using Netlify for your frontend, but you can use any static hosting provider.
Additionally, some useful flyctl commands:
flyctl logs
flyctl secrets list
flyctl ssh console
Redeploying After Wasp Builds
When you rebuild your Wasp app (with wasp build), it will remove your .wasp/build/ directory. In there, you may have a fly.toml from any prior Fly.io deployments.
While we will improve this process in the future, in the meantime, you have a few options:
Copy the fly.toml file to a versioned directory, like your Wasp project dir.
From there, you can reference it in flyctl deploy --config <path> commands, like above.
Backup the fly.toml file somewhere before running wasp build, and copy it into .wasp/build/ after.
When the fly.toml file exists in .wasp/build/ dir, you do not need to specify the --config <path>.
Run flyctl config save -a <app-name> to regenerate the fly.toml file from the remote state stored in Fly.io.
Netlify
Netlify is a static hosting solution that is free for many use cases. You will need a Netlify account and Netlify CLI installed to follow these instructions.
Make sure you are logged in with Netlify CLI. You can check if you are logged in with netlify status, and if you are not, you can log in with netlify login.
First, make sure you have built the Wasp app. We'll build the client web app next.
To build the web app, position yourself in .wasp/build/web-app directory:
Run
npm install && REACT_APP_API_URL=<url_to_wasp_backend> npm run build
where <url_to_wasp_backend> is the URL of the Wasp server that you previously deployed.
We can now deploy the client with:
Carefully follow the instructions i.e. do you want to create a new app or use an existing one, the team under which your app will reside etc.
The final step is to run:
That is it! Your client should be live at https://<app-name>.netlify.app ✨
note
Make sure you set this URL as the WASP_WEB_CLIENT_URL environment variable in your server hosting environment (e.g., Fly.io or Heroku).
Railway
Railway is a simple and great way to host your server and database. It's also possible to deploy your entire app: database, server, and client. You can use the platform for free for a limited time, or if you meet certain eligibility requirements. See their plans page for more info.
Prerequisites
To get started, follow these steps:
Make sure your Wasp app is built by running wasp build in the project dir.
Create a Railway account
Free Tier
Sign up with your GitHub account to be eligible for the free tier
Install the Railway CLI
Run railway login and a browser tab will open to authenticate you.
Create New Project
Let's create our Railway project:
Go to your Railway dashboard, click on New Project, and select Provision PostgreSQL from the dropdown menu.
Once it initializes, right-click on the New button in the top right corner and select Empty Service.
Once it initializes, click on it, go to Settings > General and change the name to server
Go ahead and create another empty service and name it client
Deploy Your App to Railway
Setup Domains
We'll need the domains for both the server and client services:
Go to the server instance's Settings tab, and click Generate Domain.
Do the same under the client's Settings.
Copy the domains as we will need them later.
Deploying the Server
Let's deploy our server first:
Move into your app's .wasp/build/ directory:
Link your app build to your newly created Railway project:
Go into the Railway dashboard and set up the required env variables:
Open the Settings and go to the Variables tab:
click Variable reference and select DATABASE_URL (it will populate it with the correct value)
add WASP_WEB_CLIENT_URL - enter the the client domain (e.g. https://client-production-XXXX.up.railway.app)
add JWT_SECRET - enter a random string at least 32 characters long (use an online generator)
Using an external auth method?
If your app is using an external authentication method(s) supported by Wasp (such as Google or GitHub), make sure to set the necessary environment variables.
Push and deploy the project:
Select server when prompted with Select Service.
Railway will now locate the Dockerfile and deploy your server 👍
Deploying the Client
Next, change into your app's frontend build directory .wasp/build/web-app:
Create the production build, using the server domain as the REACT_APP_API_URL:
npm install && REACT_APP_API_URL=<url_to_wasp_backend> npm run build
Next, we want to link this specific frontend directory to our project as well:
We need to configure Railway's static hosting for our client.
Setting Up Static Hosting
Copy the build folder within the web-app directory to dist:
We'll need to create the following files:
Dockerfile with:
Dockerfile
FROM pierrezemb/gostatic
CMD [ "-fallback", "index.html" ]
COPY ./dist/ /srv/http/
.dockerignore with:
You'll need to repeat these steps each time you run wasp build as it will remove the .wasp/build/web-app directory.
Here's a useful shell script to do the process
If you want to automate the process, save the following as deploy_client.sh in the root of your project:
deploy_client.sh
#!/usr/bin/env bash
if [ -z "$REACT_APP_API_URL" ]
then
echo "REACT_APP_API_URL is not set"
exit 1
fi
wasp build
cd .wasp/build/web-app
npm install && REACT_APP_API_URL=$REACT_APP_API_URL npm run build
cp -r build dist
dockerfile_contents=$(cat <<EOF
FROM pierrezemb/gostatic
CMD [ "-fallback", "index.html" ]
COPY ./dist/ /srv/http/
EOF
)
dockerignore_contents=$(cat <<EOF
node_modules/
EOF
)
echo "$dockerfile_contents" > Dockerfile
echo "$dockerignore_contents" > .dockerignore
railway up
Make it executable with:
chmod +x deploy_client.sh
You can run it with:
REACT_APP_API_URL=<url_to_wasp_backend> ./deploy_client.sh
Set the PORT environment variable to 8043 under the Variables tab.
Deploy the client and select client when prompted with Select Service:
Conclusion
And now your Wasp should be deployed! 🐝 🚂 🚀
Back in your Railway dashboard, click on your project and you should see your newly deployed services: Postgres, Server, and Client.
Updates & Redeploying
When you make updates and need to redeploy:
run wasp build to rebuild your app
run railway up in the .wasp/build directory (server)
repeat all the steps in the .wasp/build/web-app directory (client)
Heroku
note
Heroku used to offer free apps under certain limits. However, as of November 28, 2022, they ended support for their free tier. https://blog.heroku.com/next-chapter
As such, we recommend using an alternative provider like Fly.io for your first apps.
You will need Heroku account, heroku CLI and docker CLI installed to follow these instructions.
Make sure you are logged in with heroku CLI. You can check if you are logged in with heroku whoami, and if you are not, you can log in with heroku login.
Set Up a Heroku App
info
You need to do this only once per Wasp app.
Unless you want to deploy to an existing Heroku app, let's create a new Heroku app:
Unless you have an external Postgres database that you want to use, let's create a new database on Heroku and attach it to our app:
heroku addons:create --app <app-name> heroku-postgresql:mini
caution
Heroku does not offer a free plan anymore and mini is their cheapest database instance - it costs $5/mo.
Heroku will also set DATABASE_URL env var for us at this point. If you are using an external database, you will have to set it up yourself.
The PORT env var will also be provided by Heroku, so the only two left to set are the JWT_SECRET and WASP_WEB_CLIENT_URL env vars:
heroku config:set --app <app-name> JWT_SECRET=<random_string_at_least_32_characters_long>
heroku config:set --app <app-name> WASP_WEB_CLIENT_URL=<url_of_where_frontend_will_be_deployed>
note
If you do not know what your frontend URL is yet, don't worry. You can set WASP_WEB_CLIENT_URL after you deploy your frontend.
Deploy to a Heroku App
After you have built the app, position yourself in .wasp/build/ directory:
assuming you were at the root of your Wasp project at that moment.
Log in to Heroku Container Registry:
Build the docker image and push it to Heroku:
heroku container:push --app <app-name> web
App is still not deployed at this point. This step might take some time, especially the very first time, since there are no cached docker layers.
Note for Apple Silicon Users
Apple Silicon users need to build a non-Arm image, so the above step will not work at this time. Instead of heroku container:push, users instead should:
docker buildx build --platform linux/amd64 -t <app-name> .
docker tag <app-name> registry.heroku.com/<app-name>/web
docker push registry.heroku.com/<app-name>/web
You are now ready to proceed to the next step.
Deploy the pushed image and restart the app:
heroku container:release --app <app-name> web
This is it, the backend is deployed at https://<app-name>-XXXX.herokuapp.com 🎉
Find out the exact app URL with:
heroku info --app <app-name>
Additionally, you can check out the logs with:
heroku logs --tail --app <app-name>
Using pg-boss with Heroku
If you wish to deploy an app leveraging Jobs that use pg-boss as the executor to Heroku, you need to set an additional environment variable called PG_BOSS_NEW_OPTIONS to {"connectionString":"<REGULAR_HEROKU_DATABASE_URL>","ssl":{"rejectUnauthorized":false}}. This is because pg-boss uses the pg extension, which does not seem to connect to Heroku over SSL by default, which Heroku requires. Additionally, Heroku uses a self-signed cert, so we must handle that as well.
Read more: https://devcenter.heroku.com/articles/connecting-heroku-postgres#connecting-in-node-js | https://wasp-lang.dev/docs/0.11.8/advanced/deployment/manually |
|
2 | null | 2024-04-23T15:15:25.132Z | https://wasp-lang.dev/docs/0.11.8/advanced/deployment/cli | https://wasp-lang.dev/docs/0.11.8 | http | Wasp CLI can deploy your full-stack application with only a single command. The command automates the manual deployment process and is the recommended way of deploying Wasp apps.
## Supported Providers[](#supported-providers "Direct link to Supported Providers")
Wasp supports automated deployment to the following providers:
* [Fly.io](#flyio) - they offer 5$ free credit each month
* Railway (coming soon, track it here [#1157](https://github.com/wasp-lang/wasp/pull/1157))
## Fly.io[](#flyio "Direct link to Fly.io")
### Prerequisites[](#prerequisites "Direct link to Prerequisites")
Fly provides [free allowances](https://fly.io/docs/about/pricing/#plans) for up to 3 VMs (so deploying a Wasp app to a new account is free), but all plans require you to add your credit card information before you can proceed. If you don't, the deployment will fail.
You can add the required credit card information on the [account's billing page](https://fly.io/dashboard/personal/billing).
Fly.io CLI
You will need the [`flyctl` CLI](https://fly.io/docs/hands-on/install-flyctl/) installed on your machine before you can deploy to Fly.io.
### Deploying[](#deploying "Direct link to Deploying")
Using the Wasp CLI, you can easily deploy a new app to [Fly.io](https://fly.io/) with just a single command:
```
wasp deploy fly launch my-wasp-app mia
```
Please do not CTRL-C or exit your terminal while the commands are running.
Under the covers, this runs the equivalent of the following commands:
```
wasp deploy fly setup my-wasp-app miawasp deploy fly create-db miawasp deploy fly deploy
```
The commands above use the app basename `my-wasp-app` and deploy it to the _Miami, Florida (US) region_ (called `mia`). Read more about Fly.io regions [here](#flyio-regions).
Unique Name
Your app name must be unique across all of Fly or deployment will fail.
The basename is used to create all three app tiers, resulting in three separate apps in your Fly dashboard:
* `my-wasp-app-client`
* `my-wasp-app-server`
* `my-wasp-app-db`
You'll notice that Wasp creates two new files in your project root directory:
* `fly-server.toml`
* `fly-client.toml`
You should include these files in your version control so that you can deploy your app with a single command in the future.
### Using a Custom Domain For Your App[](#using-a-custom-domain-for-your-app "Direct link to Using a Custom Domain For Your App")
Setting up a custom domain is a three-step process:
1. You need to add your domain to your Fly client app. You can do this by running:
```
wasp deploy fly cmd --context client certs create mycoolapp.com
```
Use Your Domain
Make sure to replace `mycoolapp.com` with your domain in all of the commands mentioned in this section.
This command will output the instructions to add the DNS records to your domain. It will look something like this:
```
You can direct traffic to mycoolapp.com by:1: Adding an A record to your DNS service which reads A @ 66.241.1XX.154You can validate your ownership of mycoolapp.com by:2: Adding an AAAA record to your DNS service which reads: AAAA @ 2a09:82XX:1::1:ff40
```
2. You need to add the DNS records for your domain:
_This will depend on your domain provider, but it should be a matter of adding an A record for `@` and an AAAA record for `@` with the values provided by the previous command._
3. You need to set your domain as the `WASP_WEB_CLIENT_URL` environment variable for your server app:
```
wasp deploy fly cmd --context server secrets set WASP_WEB_CLIENT_URL=https://mycoolapp.com
```
We need to do this to keep our CORS configuration up to date.
That's it, your app should be available at `https://mycoolapp.com`! 🎉
## API Reference[](#api-reference "Direct link to API Reference")
### `launch`[](#launch "Direct link to launch")
`launch` is a convenience command that runs `setup`, `create-db`, and `deploy` in sequence.
```
wasp deploy fly launch <app-name> <region>
```
It accepts the following arguments:
* `<app-name>` - the name of your app required
* `<region>` - the region where your app will be deployed required
Read how to find the available regions [here](#flyio-regions).
It gives you the same result as running the following commands:
```
wasp deploy fly setup <app-name> <region>wasp deploy fly create-db <region>wasp deploy fly deploy
```
#### Environment Variables[](#environment-variables "Direct link to Environment Variables")
If you are deploying an app that requires any other environment variables (like social auth secrets), you can set them with the `--server-secret` option:
```
wasp deploy fly launch my-wasp-app mia --server-secret GOOGLE_CLIENT_ID=<...> --server-secret GOOGLE_CLIENT_SECRET=<...>
```
### `setup`[](#setup "Direct link to setup")
`setup` will create your client and server apps on Fly, and add some secrets, but does _not_ deploy them.
```
wasp deploy fly setup <app-name> <region>
```
It accepts the following arguments:
* `<app-name>` - the name of your app required
* `<region>` - the region where your app will be deployed required
Read how to find the available regions [here](#flyio-regions).
After running `setup`, Wasp creates two new files in your project root directory: `fly-server.toml` and `fly-client.toml`. You should include these files in your version control.
You **can edit the `fly-server.toml` and `fly-client.toml` files** to further configure your Fly deployments. Wasp will use the TOML files when you run `deploy`.
If you want to maintain multiple apps, you can add the `--fly-toml-dir <abs-path>` option to point to different directories, like "dev" or "staging".
Execute Only Once
You should only run `setup` once per app. If you run it multiple times, it will create unnecessary apps on Fly.
### `create-db`[](#create-db "Direct link to create-db")
`create-db` will create a new database for your app.
```
wasp deploy fly create-db <region>
```
It accepts the following arguments:
* `<region>` - the region where your app will be deployed required
Read how to find the available regions [here](#flyio-regions).
Execute Only Once
You should only run `create-db` once per app. If you run it multiple times, it will create multiple databases, but your app needs only one.
### `deploy`[](#deploy "Direct link to deploy")
`deploy` pushes your client and server live.
Run this command whenever you want to **update your deployed app** with the latest changes:
### `cmd`[](#cmd "Direct link to cmd")
If want to run arbitrary Fly commands (e.g. `flyctl secrets list` for your server app), here's how to do it:
```
wasp deploy fly cmd secrets list --context server
```
### Fly.io Regions[](#flyio-regions "Direct link to Fly.io Regions")
> Fly.io runs applications physically close to users: in datacenters around the world, on servers we run ourselves. You can currently deploy your apps in 34 regions, connected to a global Anycast network that makes sure your users hit our nearest server, whether they’re in Tokyo, São Paolo, or Frankfurt.
Read more on Fly regions [here](https://fly.io/docs/reference/regions/).
You can find the list of all available Fly regions by running:
#### Environment Variables[](#environment-variables-1 "Direct link to Environment Variables")
If you are deploying an app that requires any other environment variables (like social auth secrets), you can set them with the `secrets set` command:
```
wasp deploy fly cmd secrets set GOOGLE_CLIENT_ID=<...> GOOGLE_CLIENT_SECRET=<...> --context=server
```
### Mutliple Fly Organizations[](#mutliple-fly-organizations "Direct link to Mutliple Fly Organizations")
If you have multiple organizations, you can specify a `--org` option. For example:
```
wasp deploy fly launch my-wasp-app mia --org hive
```
### Building Locally[](#building-locally "Direct link to Building Locally")
Fly.io offers support for both **locally** built Docker containers and **remotely** built ones. However, for simplicity and reproducibility, the CLI defaults to the use of a remote Fly.io builder.
If you want to build locally, supply the `--build-locally` option to `wasp deploy fly launch` or `wasp deploy fly deploy`. | null | https://wasp-lang.dev/docs/0.11.8/advanced/deployment/cli | Wasp CLI can deploy your full-stack application with only a single command. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf11aa0107b3-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:25 GMT | Tue, 23 Apr 2024 15:25:25 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=f%2FSfMEmZ7VLIcxBNyX4nxfIPLv6CtN1DU24Gg4dHUjKeVPNJWOfgkAhA1h6%2Bd16AJOH64QMbaGIKskZZ5MSNaBP1rkDIlSAJw8q%2FocukU0ZCcTdhU45Yt4yukEWD5dSG"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 980c0863cad2e563cc43a6508ebb3a304633d93f | null | 3AD4:651DA:3D3A3C7:485BA16:6627D08C | null | MISS | cache-iad-kiad7000071-IAD | S1713885325.089952,VS0,VE18 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/advanced/deployment/cli | og:url | Deploying with the Wasp CLI | Wasp | og:title | Wasp CLI can deploy your full-stack application with only a single command. | og:description | Deploying with the Wasp CLI | Wasp | null | Wasp CLI can deploy your full-stack application with only a single command. The command automates the manual deployment process and is the recommended way of deploying Wasp apps.
Supported Providers
Wasp supports automated deployment to the following providers:
Fly.io - they offer 5$ free credit each month
Railway (coming soon, track it here #1157)
Fly.io
Prerequisites
Fly provides free allowances for up to 3 VMs (so deploying a Wasp app to a new account is free), but all plans require you to add your credit card information before you can proceed. If you don't, the deployment will fail.
You can add the required credit card information on the account's billing page.
Fly.io CLI
You will need the flyctl CLI installed on your machine before you can deploy to Fly.io.
Deploying
Using the Wasp CLI, you can easily deploy a new app to Fly.io with just a single command:
wasp deploy fly launch my-wasp-app mia
Please do not CTRL-C or exit your terminal while the commands are running.
Under the covers, this runs the equivalent of the following commands:
wasp deploy fly setup my-wasp-app mia
wasp deploy fly create-db mia
wasp deploy fly deploy
The commands above use the app basename my-wasp-app and deploy it to the Miami, Florida (US) region (called mia). Read more about Fly.io regions here.
Unique Name
Your app name must be unique across all of Fly or deployment will fail.
The basename is used to create all three app tiers, resulting in three separate apps in your Fly dashboard:
my-wasp-app-client
my-wasp-app-server
my-wasp-app-db
You'll notice that Wasp creates two new files in your project root directory:
fly-server.toml
fly-client.toml
You should include these files in your version control so that you can deploy your app with a single command in the future.
Using a Custom Domain For Your App
Setting up a custom domain is a three-step process:
You need to add your domain to your Fly client app. You can do this by running:
wasp deploy fly cmd --context client certs create mycoolapp.com
Use Your Domain
Make sure to replace mycoolapp.com with your domain in all of the commands mentioned in this section.
This command will output the instructions to add the DNS records to your domain. It will look something like this:
You can direct traffic to mycoolapp.com by:
1: Adding an A record to your DNS service which reads
A @ 66.241.1XX.154
You can validate your ownership of mycoolapp.com by:
2: Adding an AAAA record to your DNS service which reads:
AAAA @ 2a09:82XX:1::1:ff40
You need to add the DNS records for your domain:
This will depend on your domain provider, but it should be a matter of adding an A record for @ and an AAAA record for @ with the values provided by the previous command.
You need to set your domain as the WASP_WEB_CLIENT_URL environment variable for your server app:
wasp deploy fly cmd --context server secrets set WASP_WEB_CLIENT_URL=https://mycoolapp.com
We need to do this to keep our CORS configuration up to date.
That's it, your app should be available at https://mycoolapp.com! 🎉
API Reference
launch
launch is a convenience command that runs setup, create-db, and deploy in sequence.
wasp deploy fly launch <app-name> <region>
It accepts the following arguments:
<app-name> - the name of your app required
<region> - the region where your app will be deployed required
Read how to find the available regions here.
It gives you the same result as running the following commands:
wasp deploy fly setup <app-name> <region>
wasp deploy fly create-db <region>
wasp deploy fly deploy
Environment Variables
If you are deploying an app that requires any other environment variables (like social auth secrets), you can set them with the --server-secret option:
wasp deploy fly launch my-wasp-app mia --server-secret GOOGLE_CLIENT_ID=<...> --server-secret GOOGLE_CLIENT_SECRET=<...>
setup
setup will create your client and server apps on Fly, and add some secrets, but does not deploy them.
wasp deploy fly setup <app-name> <region>
It accepts the following arguments:
<app-name> - the name of your app required
<region> - the region where your app will be deployed required
Read how to find the available regions here.
After running setup, Wasp creates two new files in your project root directory: fly-server.toml and fly-client.toml. You should include these files in your version control.
You can edit the fly-server.toml and fly-client.toml files to further configure your Fly deployments. Wasp will use the TOML files when you run deploy.
If you want to maintain multiple apps, you can add the --fly-toml-dir <abs-path> option to point to different directories, like "dev" or "staging".
Execute Only Once
You should only run setup once per app. If you run it multiple times, it will create unnecessary apps on Fly.
create-db
create-db will create a new database for your app.
wasp deploy fly create-db <region>
It accepts the following arguments:
<region> - the region where your app will be deployed required
Read how to find the available regions here.
Execute Only Once
You should only run create-db once per app. If you run it multiple times, it will create multiple databases, but your app needs only one.
deploy
deploy pushes your client and server live.
Run this command whenever you want to update your deployed app with the latest changes:
cmd
If want to run arbitrary Fly commands (e.g. flyctl secrets list for your server app), here's how to do it:
wasp deploy fly cmd secrets list --context server
Fly.io Regions
Fly.io runs applications physically close to users: in datacenters around the world, on servers we run ourselves. You can currently deploy your apps in 34 regions, connected to a global Anycast network that makes sure your users hit our nearest server, whether they’re in Tokyo, São Paolo, or Frankfurt.
Read more on Fly regions here.
You can find the list of all available Fly regions by running:
Environment Variables
If you are deploying an app that requires any other environment variables (like social auth secrets), you can set them with the secrets set command:
wasp deploy fly cmd secrets set GOOGLE_CLIENT_ID=<...> GOOGLE_CLIENT_SECRET=<...> --context=server
Mutliple Fly Organizations
If you have multiple organizations, you can specify a --org option. For example:
wasp deploy fly launch my-wasp-app mia --org hive
Building Locally
Fly.io offers support for both locally built Docker containers and remotely built ones. However, for simplicity and reproducibility, the CLI defaults to the use of a remote Fly.io builder.
If you want to build locally, supply the --build-locally option to wasp deploy fly launch or wasp deploy fly deploy. | https://wasp-lang.dev/docs/0.11.8/advanced/deployment/cli |
|
2 | null | 2024-04-23T15:15:25.725Z | https://wasp-lang.dev/docs/0.11.8/advanced/email | https://wasp-lang.dev/docs/0.11.8 | http | ## Sending Emails
With Wasp's email sending feature, you can easily integrate email functionality into your web application.
* JavaScript
* TypeScript
main.wasp
```
app Example { ... emailSender: { provider: <provider>, defaultFrom: { name: "Example", email: "[email protected]" }, }}
```
Choose from one of the providers:
* `Mailgun`,
* `SendGrid`
* or the good old `SMTP`.
Optionally, define the `defaultFrom` field, so you don't need to provide it whenever sending an email.
## Sending Emails[](#sending-emails-1 "Direct link to Sending Emails")
Sending emails while developing
When you run your app in development mode, the emails are not sent. Instead, they are logged to the console.
To enable sending emails in development mode, you need to set the `SEND_EMAILS_IN_DEVELOPMENT` env variable to `true` in your `.env.server` file.
Before jumping into details about setting up various providers, let's see how easy it is to send emails.
You import the `emailSender` that is provided by the `@wasp/email` module and call the `send` method on it.
* JavaScript
* TypeScript
src/actions/sendEmail.js
```
import { emailSender } from "@wasp/email/index.js";// In some action handler...const info = await emailSender.send({ from: { name: "John Doe", email: "[email protected]", }, to: "[email protected]", subject: "Saying hello", text: "Hello world", html: "Hello <strong>world</strong>",});
```
Read more about the `send` method in the [API Reference](#javascript-api).
The `send` method returns an object with the status of the sent email. It varies depending on the provider you use.
## Providers[](#providers "Direct link to Providers")
For each provider, you'll need to set up env variables in the `.env.server` file at the root of your project.
### Using the SMTP Provider[](#using-the-smtp-provider "Direct link to Using the SMTP Provider")
First, set the provider to `SMTP` in your `main.wasp` file.
* JavaScript
* TypeScript
main.wasp
```
app Example { ... emailSender: { provider: SMTP, }}
```
Then, add the following env variables to your `.env.server` file.
.env.server
```
SMTP_HOST=SMTP_USERNAME=SMTP_PASSWORD=SMTP_PORT=
```
Many transactional email providers (e.g. Mailgun, SendGrid but also others) can also use SMTP, so you can use them as well.
### Using the Mailgun Provider[](#using-the-mailgun-provider "Direct link to Using the Mailgun Provider")
Set the provider to `Mailgun` in the `main.wasp` file.
* JavaScript
* TypeScript
main.wasp
```
app Example { ... emailSender: { provider: Mailgun, }}
```
Then, get the Mailgun API key and domain and add them to your `.env.server` file.
#### Getting the API Key and Domain[](#getting-the-api-key-and-domain "Direct link to Getting the API Key and Domain")
1. Go to [Mailgun](https://www.mailgun.com/) and create an account.
2. Go to [API Keys](https://app.mailgun.com/app/account/security/api_keys) and create a new API key.
3. Copy the API key and add it to your `.env.server` file.
4. Go to [Domains](https://app.mailgun.com/app/domains) and create a new domain.
5. Copy the domain and add it to your `.env.server` file.
.env.server
```
MAILGUN_API_KEY=MAILGUN_DOMAIN=
```
### Using the SendGrid Provider[](#using-the-sendgrid-provider "Direct link to Using the SendGrid Provider")
Set the provider field to `SendGrid` in your `main.wasp` file.
* JavaScript
* TypeScript
main.wasp
```
app Example { ... emailSender: { provider: SendGrid, }}
```
Then, get the SendGrid API key and add it to your `.env.server` file.
#### Getting the API Key[](#getting-the-api-key "Direct link to Getting the API Key")
1. Go to [SendGrid](https://sendgrid.com/) and create an account.
2. Go to [API Keys](https://app.sendgrid.com/settings/api_keys) and create a new API key.
3. Copy the API key and add it to your `.env.server` file.
## API Reference[](#api-reference "Direct link to API Reference")
### `emailSender` dict[](#emailsender-dict "Direct link to emailsender-dict")
* JavaScript
* TypeScript
main.wasp
```
app Example { ... emailSender: { provider: <provider>, defaultFrom: { name: "Example", email: "[email protected]" }, }}
```
The `emailSender` dict has the following fields:
* `provider: Provider` required
The provider you want to use. Choose from `SMTP`, `Mailgun` or `SendGrid`.
* `defaultFrom: dict`
The default sender's details. If you set this field, you don't need to provide the `from` field when sending an email.
### JavaScript API[](#javascript-api "Direct link to JavaScript API")
Using the `emailSender` in :
* JavaScript
* TypeScript
src/actions/sendEmail.js
```
import { emailSender } from "@wasp/email/index.js";// In some action handler...const info = await emailSender.send({ from: { name: "John Doe", email: "[email protected]", }, to: "[email protected]", subject: "Saying hello", text: "Hello world", html: "Hello <strong>world</strong>",});
```
The `send` method accepts an object with the following fields:
* `from: object`
The sender's details. If you set up `defaultFrom` field in the `emailSender` dict in Wasp file, this field is optional.
* `name: string`
The name of the sender.
* `email: string`
The email address of the sender.
* `to: string` required
The recipient's email address.
* `subject: string` required
The subject of the email.
* `text: string` required
The text version of the email.
* `html: string` required
The HTML version of the email | null | https://wasp-lang.dev/docs/0.11.8/advanced/email | With Wasp's email sending feature, you can easily integrate email functionality into your web application. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf1559c959cd-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:25 GMT | Tue, 23 Apr 2024 15:25:25 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=0ptlrhW7EDfCcARWLqe4fzfBuC0YRDqNl%2FFzxKZJTEp4sPhkgchzwBtf0lqckZlwJf9RlSzl0bHWP5JcAAD2wIOEgrydD05BSDit%2BGHU6pY%2FsmKfwzPaF4NWQP%2FUJtuM"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 6bcdee76a7c801a641d59f92b45d3ff510b0e45c | null | 83C8:1A89F:3BDB9F4:46E1B8F:6627D08D | null | MISS | cache-iad-kiad7000071-IAD | S1713885326.670299,VS0,VE16 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/advanced/email | og:url | Sending Emails | Wasp | og:title | With Wasp's email sending feature, you can easily integrate email functionality into your web application. | og:description | Sending Emails | Wasp | null | Sending Emails
With Wasp's email sending feature, you can easily integrate email functionality into your web application.
JavaScript
TypeScript
main.wasp
app Example {
...
emailSender: {
provider: <provider>,
defaultFrom: {
name: "Example",
email: "[email protected]"
},
}
}
Choose from one of the providers:
Mailgun,
SendGrid
or the good old SMTP.
Optionally, define the defaultFrom field, so you don't need to provide it whenever sending an email.
Sending Emails
Sending emails while developing
When you run your app in development mode, the emails are not sent. Instead, they are logged to the console.
To enable sending emails in development mode, you need to set the SEND_EMAILS_IN_DEVELOPMENT env variable to true in your .env.server file.
Before jumping into details about setting up various providers, let's see how easy it is to send emails.
You import the emailSender that is provided by the @wasp/email module and call the send method on it.
JavaScript
TypeScript
src/actions/sendEmail.js
import { emailSender } from "@wasp/email/index.js";
// In some action handler...
const info = await emailSender.send({
from: {
name: "John Doe",
email: "[email protected]",
},
to: "[email protected]",
subject: "Saying hello",
text: "Hello world",
html: "Hello <strong>world</strong>",
});
Read more about the send method in the API Reference.
The send method returns an object with the status of the sent email. It varies depending on the provider you use.
Providers
For each provider, you'll need to set up env variables in the .env.server file at the root of your project.
Using the SMTP Provider
First, set the provider to SMTP in your main.wasp file.
JavaScript
TypeScript
main.wasp
app Example {
...
emailSender: {
provider: SMTP,
}
}
Then, add the following env variables to your .env.server file.
.env.server
SMTP_HOST=
SMTP_USERNAME=
SMTP_PASSWORD=
SMTP_PORT=
Many transactional email providers (e.g. Mailgun, SendGrid but also others) can also use SMTP, so you can use them as well.
Using the Mailgun Provider
Set the provider to Mailgun in the main.wasp file.
JavaScript
TypeScript
main.wasp
app Example {
...
emailSender: {
provider: Mailgun,
}
}
Then, get the Mailgun API key and domain and add them to your .env.server file.
Getting the API Key and Domain
Go to Mailgun and create an account.
Go to API Keys and create a new API key.
Copy the API key and add it to your .env.server file.
Go to Domains and create a new domain.
Copy the domain and add it to your .env.server file.
.env.server
MAILGUN_API_KEY=
MAILGUN_DOMAIN=
Using the SendGrid Provider
Set the provider field to SendGrid in your main.wasp file.
JavaScript
TypeScript
main.wasp
app Example {
...
emailSender: {
provider: SendGrid,
}
}
Then, get the SendGrid API key and add it to your .env.server file.
Getting the API Key
Go to SendGrid and create an account.
Go to API Keys and create a new API key.
Copy the API key and add it to your .env.server file.
API Reference
emailSender dict
JavaScript
TypeScript
main.wasp
app Example {
...
emailSender: {
provider: <provider>,
defaultFrom: {
name: "Example",
email: "[email protected]"
},
}
}
The emailSender dict has the following fields:
provider: Provider required
The provider you want to use. Choose from SMTP, Mailgun or SendGrid.
defaultFrom: dict
The default sender's details. If you set this field, you don't need to provide the from field when sending an email.
JavaScript API
Using the emailSender in :
JavaScript
TypeScript
src/actions/sendEmail.js
import { emailSender } from "@wasp/email/index.js";
// In some action handler...
const info = await emailSender.send({
from: {
name: "John Doe",
email: "[email protected]",
},
to: "[email protected]",
subject: "Saying hello",
text: "Hello world",
html: "Hello <strong>world</strong>",
});
The send method accepts an object with the following fields:
from: object
The sender's details. If you set up defaultFrom field in the emailSender dict in Wasp file, this field is optional.
name: string
The name of the sender.
email: string
The email address of the sender.
to: string required
The recipient's email address.
subject: string required
The subject of the email.
text: string required
The text version of the email.
html: string required
The HTML version of the email | https://wasp-lang.dev/docs/0.11.8/advanced/email |
|
2 | null | 2024-04-23T15:15:27.483Z | https://wasp-lang.dev/docs/0.11.8/advanced/web-sockets | https://wasp-lang.dev/docs/0.11.8 | http | ## Web Sockets
Wasp provides a fully integrated WebSocket experience by utilizing [Socket.IO](https://socket.io/) on the client and server.
We handle making sure your URLs are correctly setup, CORS is enabled, and provide a useful `useSocket` and `useSocketListener` abstractions for use in React components.
To get started, you need to:
1. Define your WebSocket logic on the server.
2. Enable WebSockets in your Wasp file, and connect it with your server logic.
3. Use WebSockets on the client, in React, via `useSocket` and `useSocketListener`.
4. Optionally, type the WebSocket events and payloads for full-stack type safety.
Let's go through setting up WebSockets step by step, starting with enabling WebSockets in your Wasp file.
## Turn On WebSockets in Your Wasp File[](#turn-on-websockets-in-your-wasp-file "Direct link to Turn On WebSockets in Your Wasp File")
We specify that we are using WebSockets by adding `webSocket` to our `app` and providing the required `fn`. You can optionally change the auto-connect behavior.
* JavaScript
* TypeScript
todoApp.wasp
```
app todoApp { // ... webSocket: { fn: import { webSocketFn } from "@server/webSocket.js", autoConnect: true, // optional, default: true },}
```
## Defining the Events Handler[](#defining-the-events-handler "Direct link to Defining the Events Handler")
Let's define the WebSockets server with all of the events and handler functions.
### `webSocketFn` Function[](#websocketfn-function "Direct link to websocketfn-function")
On the server, you will get Socket.IO `io: Server` argument and `context` for your WebSocket function. The `context` object give you access to all of the entities from your Wasp app.
You can use this `io` object to register callbacks for all the regular [Socket.IO events](https://socket.io/docs/v4/server-api/). Also, if a user is logged in, you will have a `socket.data.user` on the server.
This is how we can define our `webSocketFn` function:
* JavaScript
* TypeScript
src/server/webSocket.js
```
import { v4 as uuidv4 } from 'uuid'export const webSocketFn = (io, context) => { io.on('connection', (socket) => { const username = socket.data.user?.email || socket.data.user?.username || 'unknown' console.log('a user connected: ', username) socket.on('chatMessage', async (msg) => { console.log('message: ', msg) io.emit('chatMessage', { id: uuidv4(), username, text: msg }) // You can also use your entities here: // await context.entities.SomeEntity.create({ someField: msg }) }) })}
```
## Using the WebSocket On The Client[](#using-the-websocket-on-the-client "Direct link to Using the WebSocket On The Client")
### `useSocket` Hook[](#usesocket-hook "Direct link to usesocket-hook")
Client access to WebSockets is provided by the `useSocket` hook. It returns:
* `socket: Socket` for sending and receiving events.
* `isConnected: boolean` for showing a display of the Socket.IO connection status.
* Note: Wasp automatically connects and establishes a WebSocket connection from the client to the server by default, so you do not need to explicitly `socket.connect()` or `socket.disconnect()`.
* If you set `autoConnect: false` in your Wasp file, then you should call these as needed.
All components using `useSocket` share the same underlying `socket`.
### `useSocketListener` Hook[](#usesocketlistener-hook "Direct link to usesocketlistener-hook")
Additionally, there is a `useSocketListener: (event, callback) => void` hook which is used for registering event handlers. It takes care of unregistering the handler on unmount.
* JavaScript
* TypeScript
src/client/ChatPage.jsx
```
import React, { useState } from 'react'import { useSocket, useSocketListener,} from '@wasp/webSocket'export const ChatPage = () => { const [messageText, setMessageText] = useState('') const [messages, setMessages] = useState([]) const { socket, isConnected } = useSocket() useSocketListener('chatMessage', logMessage) function logMessage(msg) { setMessages((priorMessages) => [msg, ...priorMessages]) } function handleSubmit(e) { e.preventDefault() socket.emit('chatMessage', messageText) setMessageText('') } const messageList = messages.map((msg) => ( <li key={msg.id}> <em>{msg.username}</em>: {msg.text} </li> )) const connectionIcon = isConnected ? '🟢' : '🔴' return ( <> <h2>Chat {connectionIcon}</h2> <div> <form onSubmit={handleSubmit}> <div> <div> <input type="text" value={messageText} onChange={(e) => setMessageText(e.target.value)} /> </div> <div> <button type="submit">Submit</button> </div> </div> </form> <ul>{messageList}</ul> </div> </> )}
```
## API Reference[](#api-reference "Direct link to API Reference")
* JavaScript
* TypeScript
todoApp.wasp
```
app todoApp { // ... webSocket: { fn: import { webSocketFn } from "@server/webSocket.js", autoConnect: true, // optional, default: true },}
```
The `webSocket` dict has the following fields:
* `fn: WebSocketFn` required
The function that defines the WebSocket events and handlers.
* `autoConnect: bool`
Whether to automatically connect to the WebSocket server. Default: `true`. | null | https://wasp-lang.dev/docs/0.11.8/advanced/web-sockets | Wasp provides a fully integrated WebSocket experience by utilizing Socket.IO on the client and server. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf204c1e07a0-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:27 GMT | Tue, 23 Apr 2024 15:25:27 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=zTQocJbBaffLq82bCwoNHEqEzyyPIDbnv2z77YGJsyfNN0i%2Fju61p5JuZ%2BYQHY69oPBOWQO2ei3leL9iBxlikxi%2BcdTkDoU0V1Pl4WqeCxzvL%2BwgQ%2F08WtYtAQ4TdwIn"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 9199816e5daa2fa592476faf2de8341f9bdc9f70 | null | D10A:2C5630:3C56BE4:475C952:6627D08E | HIT | MISS | cache-iad-kiad7000118-IAD | S1713885327.435949,VS0,VE12 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/advanced/web-sockets | og:url | Web Sockets | Wasp | og:title | Wasp provides a fully integrated WebSocket experience by utilizing Socket.IO on the client and server. | og:description | Web Sockets | Wasp | null | Web Sockets
Wasp provides a fully integrated WebSocket experience by utilizing Socket.IO on the client and server.
We handle making sure your URLs are correctly setup, CORS is enabled, and provide a useful useSocket and useSocketListener abstractions for use in React components.
To get started, you need to:
Define your WebSocket logic on the server.
Enable WebSockets in your Wasp file, and connect it with your server logic.
Use WebSockets on the client, in React, via useSocket and useSocketListener.
Optionally, type the WebSocket events and payloads for full-stack type safety.
Let's go through setting up WebSockets step by step, starting with enabling WebSockets in your Wasp file.
Turn On WebSockets in Your Wasp File
We specify that we are using WebSockets by adding webSocket to our app and providing the required fn. You can optionally change the auto-connect behavior.
JavaScript
TypeScript
todoApp.wasp
app todoApp {
// ...
webSocket: {
fn: import { webSocketFn } from "@server/webSocket.js",
autoConnect: true, // optional, default: true
},
}
Defining the Events Handler
Let's define the WebSockets server with all of the events and handler functions.
webSocketFn Function
On the server, you will get Socket.IO io: Server argument and context for your WebSocket function. The context object give you access to all of the entities from your Wasp app.
You can use this io object to register callbacks for all the regular Socket.IO events. Also, if a user is logged in, you will have a socket.data.user on the server.
This is how we can define our webSocketFn function:
JavaScript
TypeScript
src/server/webSocket.js
import { v4 as uuidv4 } from 'uuid'
export const webSocketFn = (io, context) => {
io.on('connection', (socket) => {
const username = socket.data.user?.email || socket.data.user?.username || 'unknown'
console.log('a user connected: ', username)
socket.on('chatMessage', async (msg) => {
console.log('message: ', msg)
io.emit('chatMessage', { id: uuidv4(), username, text: msg })
// You can also use your entities here:
// await context.entities.SomeEntity.create({ someField: msg })
})
})
}
Using the WebSocket On The Client
useSocket Hook
Client access to WebSockets is provided by the useSocket hook. It returns:
socket: Socket for sending and receiving events.
isConnected: boolean for showing a display of the Socket.IO connection status.
Note: Wasp automatically connects and establishes a WebSocket connection from the client to the server by default, so you do not need to explicitly socket.connect() or socket.disconnect().
If you set autoConnect: false in your Wasp file, then you should call these as needed.
All components using useSocket share the same underlying socket.
useSocketListener Hook
Additionally, there is a useSocketListener: (event, callback) => void hook which is used for registering event handlers. It takes care of unregistering the handler on unmount.
JavaScript
TypeScript
src/client/ChatPage.jsx
import React, { useState } from 'react'
import {
useSocket,
useSocketListener,
} from '@wasp/webSocket'
export const ChatPage = () => {
const [messageText, setMessageText] = useState('')
const [messages, setMessages] = useState([])
const { socket, isConnected } = useSocket()
useSocketListener('chatMessage', logMessage)
function logMessage(msg) {
setMessages((priorMessages) => [msg, ...priorMessages])
}
function handleSubmit(e) {
e.preventDefault()
socket.emit('chatMessage', messageText)
setMessageText('')
}
const messageList = messages.map((msg) => (
<li key={msg.id}>
<em>{msg.username}</em>: {msg.text}
</li>
))
const connectionIcon = isConnected ? '🟢' : '🔴'
return (
<>
<h2>Chat {connectionIcon}</h2>
<div>
<form onSubmit={handleSubmit}>
<div>
<div>
<input
type="text"
value={messageText}
onChange={(e) => setMessageText(e.target.value)}
/>
</div>
<div>
<button type="submit">Submit</button>
</div>
</div>
</form>
<ul>{messageList}</ul>
</div>
</>
)
}
API Reference
JavaScript
TypeScript
todoApp.wasp
app todoApp {
// ...
webSocket: {
fn: import { webSocketFn } from "@server/webSocket.js",
autoConnect: true, // optional, default: true
},
}
The webSocket dict has the following fields:
fn: WebSocketFn required
The function that defines the WebSocket events and handlers.
autoConnect: bool
Whether to automatically connect to the WebSocket server. Default: true. | https://wasp-lang.dev/docs/0.11.8/advanced/web-sockets |
|
2 | null | 2024-04-23T15:15:27.449Z | https://wasp-lang.dev/docs/0.11.8/advanced/jobs | https://wasp-lang.dev/docs/0.11.8 | http | Version: 0.11.8
## Recurring Jobs
In most web apps, users send requests to the server and receive responses with some data. When the server responds quickly, the app feels responsive and smooth.
What if the server needs extra time to fully process the request? This might mean sending an email or making a slow HTTP request to an external API. In that case, it's a good idea to respond to the user as soon as possible and do the remaining work in the background.
Wasp supports background jobs that can help you with this:
* Jobs persist between server restarts,
* Jobs can be retried if they fail,
* Jobs can be delayed until a future time,
* Jobs can have a recurring schedule.
## Using Jobs[](#using-jobs "Direct link to Using Jobs")
### Job Definition and Usage[](#job-definition-and-usage "Direct link to Job Definition and Usage")
Let's write an example Job that will print a message to the console and return a list of tasks from the database.
1. Start by creating a Job declaration in your `.wasp` file:
* JavaScript
* TypeScript
main.wasp
```
job mySpecialJob { executor: PgBoss, perform: { fn: import { foo } from "@server/workers/bar.js" }, entities: [Task],}
```
2. After declaring the Job, implement its worker function:
* JavaScript
* TypeScript
bar.js
```
export const foo = async ({ name }, context) => { console.log(`Hello ${name}!`) const tasks = await context.entities.Task.findMany({}) return { tasks }}
```
The worker function
The worker function must be an `async` function. The function's return value represents the Job's result.
The worker function accepts two arguments:
* `args`: The data passed into the job when it's submitted.
* `context: { entities }`: The context object containing entities you put in the Job declaration.
3. After successfully defining the job, you can submit work to be done in your [Operations](https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview) or [setupFn](https://wasp-lang.dev/docs/0.11.8/project/server-config#setup-function) (or any other NodeJS code):
* JavaScript
* TypeScript
someAction.js
```
import { mySpecialJob } from '@wasp/jobs/mySpecialJob.js'const submittedJob = await mySpecialJob.submit({ job: "Johnny" })// Or, if you'd prefer it to execute in the future, just add a .delay().// It takes a number of seconds, Date, or ISO date string.await mySpecialJob .delay(10) .submit({ name: "Johnny" })
```
And that'is it. Your job will be executed by `PgBoss` as if you called `foo({ name: "Johnny" })`.
In our example, `foo` takes an argument, but passing arguments to jobs is not a requirement. It depends on how you've implemented your worker function.
### Recurring Jobs[](#recurring-jobs "Direct link to Recurring Jobs")
If you have work that needs to be done on some recurring basis, you can add a `schedule` to your job declaration:
* JavaScript
* TypeScript
main.wasp
```
job mySpecialJob { executor: PgBoss, perform: { fn: import { foo } from "@server/workers/bar.js" }, schedule: { cron: "0 * * * *", args: {=json { "job": "args" } json=} // optional }}
```
In this example, you _don't_ need to invoke anything in . You can imagine `foo({ job: "args" })` getting automatically scheduled and invoked for you every hour.
## API Reference[](#api-reference "Direct link to API Reference")
### Declaring Jobs[](#declaring-jobs "Direct link to Declaring Jobs")
* JavaScript
* TypeScript
main.wasp
```
job mySpecialJob { executor: PgBoss, perform: { fn: import { foo } from "@server/workers/bar.js", executorOptions: { pgBoss: {=json { "retryLimit": 1 } json=} } }, schedule: { cron: "*/5 * * * *", args: {=json { "foo": "bar" } json=}, executorOptions: { pgBoss: {=json { "retryLimit": 0 } json=} } }, entities: [Task],}
```
The Job declaration has the following fields:
* `executor: JobExecutor` required
Job executors
Our jobs need job executors to handle the _scheduling, monitoring, and execution_.
`PgBoss` is currently our only job executor, and is recommended for low-volume production use cases. It requires your `app.db.system` to be `PostgreSQL`.
We have selected [pg-boss](https://github.com/timgit/pg-boss/) as our first job executor to handle the low-volume, basic job queue workloads many web applications have. By using PostgreSQL (and [SKIP LOCKED](https://www.2ndquadrant.com/en/blog/what-is-select-skip-locked-for-in-postgresql-9-5/)) as its storage and synchronization mechanism, it allows us to provide many job queue pros without any additional infrastructure or complex management.
info
Keep in mind that pg-boss jobs run alongside your other server-side code, so they are not appropriate for CPU-heavy workloads. Additionally, some care is required if you modify scheduled jobs. Please see pg-boss details below for more information.
pg-boss details
pg-boss provides many useful features, which can be found [here](https://github.com/timgit/pg-boss/blob/8.4.2/README.md).
When you add pg-boss to a Wasp project, it will automatically add a new schema to your database called `pgboss` with some internal tracking tables, including `job` and `schedule`. pg-boss tables have a `name` column in most tables that will correspond to your Job identifier. Additionally, these tables maintain arguments, states, return values, retry information, start and expiration times, and other metadata required by pg-boss.
If you need to customize the creation of the pg-boss instance, you can set an environment variable called `PG_BOSS_NEW_OPTIONS` to a stringified JSON object containing [these initialization parameters](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#newoptions). **NOTE**: Setting this overwrites all Wasp defaults, so you must include database connection information as well.
### pg-boss considerations[](#pg-boss-considerations "Direct link to pg-boss considerations")
* Wasp starts pg-boss alongside your web server's application, where both are simultaneously operational. This means that jobs running via pg-boss and the rest of the server logic (like Operations) share the CPU, therefore you should avoid running CPU-intensive tasks via jobs.
* Wasp does not (yet) support independent, horizontal scaling of pg-boss-only applications, nor starting them as separate workers/processes/threads.
* The job name/identifier in your `.wasp` file is the same name that will be used in the `name` column of pg-boss tables. If you change a name that had a `schedule` associated with it, pg-boss will continue scheduling those jobs but they will have no handlers associated, and will thus become stale and expire. To resolve this, you can remove the applicable row from the `schedule` table in the `pgboss` schema of your database.
* If you remove a `schedule` from a job, you will need to do the above as well.
* If you wish to deploy to Heroku, you need to set an additional environment variable called `PG_BOSS_NEW_OPTIONS` to `{"connectionString":"<REGULAR_HEROKU_DATABASE_URL>","ssl":{"rejectUnauthorized":false}}`. This is because pg-boss uses the `pg` extension, which does not seem to connect to Heroku over SSL by default, which Heroku requires. Additionally, Heroku uses a self-signed cert, so we must handle that as well.
* [https://devcenter.heroku.com/articles/connecting-heroku-postgres#connecting-in-node-js](https://devcenter.heroku.com/articles/connecting-heroku-postgres#connecting-in-node-js)
* `perform: dict` required
* `fn: ServerImport` required
* An `async` function that performs the work. Since Wasp executes Jobs on the server, you must import it from `@server`.
* It receives the following arguments:
* `args: Input`: The data passed to the job when it's submitted.
* `context: { entities: Entities }`: The context object containing any declared entities.
Here's an example of a `perform.fn` function:
* JavaScript
* TypeScript
bar.js
```
export const foo = async ({ name }, context) => { console.log(`Hello ${name}!`) const tasks = await context.entities.Task.findMany({}) return { tasks }}
```
* `executorOptions: dict`
Executor-specific default options to use when submitting jobs. These are passed directly through and you should consult the documentation for the job executor. These can be overridden during invocation with `submit()` or in a `schedule`.
* `pgBoss: JSON`
See the docs for [pg-boss](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#sendname-data-options).
* `schedule: dict`
* `cron: string` required
A 5-placeholder format cron expression string. See rationale for minute-level precision [here](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#scheduling).
_If you need help building cron expressions, Check out_ _[Crontab guru](https://crontab.guru/#0_*_*_*_*)._
* `args: JSON`
The arguments to pass to the `perform.fn` function when invoked.
* `executorOptions: dict`
Executor-specific options to use when submitting jobs. These are passed directly through and you should consult the documentation for the job executor. The `perform.executorOptions` are the default options, and `schedule.executorOptions` can override/extend those.
* `pgBoss: JSON`
See the docs for [pg-boss](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#sendname-data-options).
* `entities: [Entity]`
A list of entities you wish to use inside your Job (similar to [Queries and Actions](https://wasp-lang.dev/docs/0.11.8/data-model/operations/queries#using-entities-in-queries)).
### JavaScript API[](#javascript-api "Direct link to JavaScript API")
* Importing a Job:
* JavaScript
* TypeScript
someAction.js
```
import { mySpecialJob } from '@wasp/jobs/mySpecialJob.js'
```
* `submit(jobArgs, executorOptions)`
* `jobArgs: Input`
* `executorOptions: object`
Submits a Job to be executed by an executor, optionally passing in a JSON job argument your job handler function receives, and executor-specific submit options.
* JavaScript
* TypeScript
someAction.js
```
const submittedJob = await mySpecialJob.submit({ job: "args" })
```
* `delay(startAfter)`
* `startAfter: int | string | Date` required
Delaying the invocation of the job handler. The delay can be one of:
* Integer: number of seconds to delay. \[Default 0\]
* String: ISO date string to run at.
* Date: Date to run at.
* JavaScript
* TypeScript
someAction.js
```
const submittedJob = await mySpecialJob .delay(10) .submit({ job: "args" }, { "retryLimit": 2 })
```
#### Tracking[](#tracking "Direct link to Tracking")
The return value of `submit()` is an instance of `SubmittedJob`, which has the following fields:
* `jobId`: The ID for the job in that executor.
* `jobName`: The name of the job you used in your `.wasp` file.
* `executorName`: The Symbol of the name of the job executor.
* For pg-boss, you can import a Symbol from: `import { PG_BOSS_EXECUTOR_NAME } from '@wasp/jobs/core/pgBoss/pgBossJob.js'` if you wish to compare against `executorName`.
There are also some namespaced, job executor-specific objects.
* For pg-boss, you may access: `pgBoss`
* `details()`: pg-boss specific job detail information. [Reference](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#getjobbyidid)
* `cancel()`: attempts to cancel a job. [Reference](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#cancelid)
* `resume()`: attempts to resume a canceled job. [Reference](https://github.com/timgit/pg-boss/blob/8.4.2/docs/readme.md#resumeid) | null | https://wasp-lang.dev/docs/0.11.8/advanced/jobs | In most web apps, users send requests to the server and receive responses with some data. When the server responds quickly, the app feels responsive and smooth. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf1ffd4d397a-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:27 GMT | Tue, 23 Apr 2024 15:25:27 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=mwPXn16FVtA9PPIK8XQm1xvrGKWZU0GNszOSGI0IU57rTjhMg%2F%2F4rf8jUw%2FB%2F09MuKNGqz4xh%2BSZ92fTjZ9FQ%2F4xnCM60cKnqjBSnrgHvDydADs4omJKYTDPfDSi4zPe"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 5a09be569e56e7a70e833eff6b9deeddc8508c30 | null | DD9C:180046:3C2FFCD:473674D:6627D08E | HIT | MISS | cache-iad-kiad7000111-IAD | S1713885327.398901,VS0,VE14 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/advanced/jobs | og:url | Recurring Jobs | Wasp | og:title | In most web apps, users send requests to the server and receive responses with some data. When the server responds quickly, the app feels responsive and smooth. | og:description | Recurring Jobs | Wasp | null | Version: 0.11.8
Recurring Jobs
In most web apps, users send requests to the server and receive responses with some data. When the server responds quickly, the app feels responsive and smooth.
What if the server needs extra time to fully process the request? This might mean sending an email or making a slow HTTP request to an external API. In that case, it's a good idea to respond to the user as soon as possible and do the remaining work in the background.
Wasp supports background jobs that can help you with this:
Jobs persist between server restarts,
Jobs can be retried if they fail,
Jobs can be delayed until a future time,
Jobs can have a recurring schedule.
Using Jobs
Job Definition and Usage
Let's write an example Job that will print a message to the console and return a list of tasks from the database.
Start by creating a Job declaration in your .wasp file:
JavaScript
TypeScript
main.wasp
job mySpecialJob {
executor: PgBoss,
perform: {
fn: import { foo } from "@server/workers/bar.js"
},
entities: [Task],
}
After declaring the Job, implement its worker function:
JavaScript
TypeScript
bar.js
export const foo = async ({ name }, context) => {
console.log(`Hello ${name}!`)
const tasks = await context.entities.Task.findMany({})
return { tasks }
}
The worker function
The worker function must be an async function. The function's return value represents the Job's result.
The worker function accepts two arguments:
args: The data passed into the job when it's submitted.
context: { entities }: The context object containing entities you put in the Job declaration.
After successfully defining the job, you can submit work to be done in your Operations or setupFn (or any other NodeJS code):
JavaScript
TypeScript
someAction.js
import { mySpecialJob } from '@wasp/jobs/mySpecialJob.js'
const submittedJob = await mySpecialJob.submit({ job: "Johnny" })
// Or, if you'd prefer it to execute in the future, just add a .delay().
// It takes a number of seconds, Date, or ISO date string.
await mySpecialJob
.delay(10)
.submit({ name: "Johnny" })
And that'is it. Your job will be executed by PgBoss as if you called foo({ name: "Johnny" }).
In our example, foo takes an argument, but passing arguments to jobs is not a requirement. It depends on how you've implemented your worker function.
Recurring Jobs
If you have work that needs to be done on some recurring basis, you can add a schedule to your job declaration:
JavaScript
TypeScript
main.wasp
job mySpecialJob {
executor: PgBoss,
perform: {
fn: import { foo } from "@server/workers/bar.js"
},
schedule: {
cron: "0 * * * *",
args: {=json { "job": "args" } json=} // optional
}
}
In this example, you don't need to invoke anything in . You can imagine foo({ job: "args" }) getting automatically scheduled and invoked for you every hour.
API Reference
Declaring Jobs
JavaScript
TypeScript
main.wasp
job mySpecialJob {
executor: PgBoss,
perform: {
fn: import { foo } from "@server/workers/bar.js",
executorOptions: {
pgBoss: {=json { "retryLimit": 1 } json=}
}
},
schedule: {
cron: "*/5 * * * *",
args: {=json { "foo": "bar" } json=},
executorOptions: {
pgBoss: {=json { "retryLimit": 0 } json=}
}
},
entities: [Task],
}
The Job declaration has the following fields:
executor: JobExecutor required
Job executors
Our jobs need job executors to handle the scheduling, monitoring, and execution.
PgBoss is currently our only job executor, and is recommended for low-volume production use cases. It requires your app.db.system to be PostgreSQL.
We have selected pg-boss as our first job executor to handle the low-volume, basic job queue workloads many web applications have. By using PostgreSQL (and SKIP LOCKED) as its storage and synchronization mechanism, it allows us to provide many job queue pros without any additional infrastructure or complex management.
info
Keep in mind that pg-boss jobs run alongside your other server-side code, so they are not appropriate for CPU-heavy workloads. Additionally, some care is required if you modify scheduled jobs. Please see pg-boss details below for more information.
pg-boss details
pg-boss provides many useful features, which can be found here.
When you add pg-boss to a Wasp project, it will automatically add a new schema to your database called pgboss with some internal tracking tables, including job and schedule. pg-boss tables have a name column in most tables that will correspond to your Job identifier. Additionally, these tables maintain arguments, states, return values, retry information, start and expiration times, and other metadata required by pg-boss.
If you need to customize the creation of the pg-boss instance, you can set an environment variable called PG_BOSS_NEW_OPTIONS to a stringified JSON object containing these initialization parameters. NOTE: Setting this overwrites all Wasp defaults, so you must include database connection information as well.
pg-boss considerations
Wasp starts pg-boss alongside your web server's application, where both are simultaneously operational. This means that jobs running via pg-boss and the rest of the server logic (like Operations) share the CPU, therefore you should avoid running CPU-intensive tasks via jobs.
Wasp does not (yet) support independent, horizontal scaling of pg-boss-only applications, nor starting them as separate workers/processes/threads.
The job name/identifier in your .wasp file is the same name that will be used in the name column of pg-boss tables. If you change a name that had a schedule associated with it, pg-boss will continue scheduling those jobs but they will have no handlers associated, and will thus become stale and expire. To resolve this, you can remove the applicable row from the schedule table in the pgboss schema of your database.
If you remove a schedule from a job, you will need to do the above as well.
If you wish to deploy to Heroku, you need to set an additional environment variable called PG_BOSS_NEW_OPTIONS to {"connectionString":"<REGULAR_HEROKU_DATABASE_URL>","ssl":{"rejectUnauthorized":false}}. This is because pg-boss uses the pg extension, which does not seem to connect to Heroku over SSL by default, which Heroku requires. Additionally, Heroku uses a self-signed cert, so we must handle that as well.
https://devcenter.heroku.com/articles/connecting-heroku-postgres#connecting-in-node-js
perform: dict required
fn: ServerImport required
An async function that performs the work. Since Wasp executes Jobs on the server, you must import it from @server.
It receives the following arguments:
args: Input: The data passed to the job when it's submitted.
context: { entities: Entities }: The context object containing any declared entities.
Here's an example of a perform.fn function:
JavaScript
TypeScript
bar.js
export const foo = async ({ name }, context) => {
console.log(`Hello ${name}!`)
const tasks = await context.entities.Task.findMany({})
return { tasks }
}
executorOptions: dict
Executor-specific default options to use when submitting jobs. These are passed directly through and you should consult the documentation for the job executor. These can be overridden during invocation with submit() or in a schedule.
pgBoss: JSON
See the docs for pg-boss.
schedule: dict
cron: string required
A 5-placeholder format cron expression string. See rationale for minute-level precision here.
If you need help building cron expressions, Check out Crontab guru.
args: JSON
The arguments to pass to the perform.fn function when invoked.
executorOptions: dict
Executor-specific options to use when submitting jobs. These are passed directly through and you should consult the documentation for the job executor. The perform.executorOptions are the default options, and schedule.executorOptions can override/extend those.
pgBoss: JSON
See the docs for pg-boss.
entities: [Entity]
A list of entities you wish to use inside your Job (similar to Queries and Actions).
JavaScript API
Importing a Job:
JavaScript
TypeScript
someAction.js
import { mySpecialJob } from '@wasp/jobs/mySpecialJob.js'
submit(jobArgs, executorOptions)
jobArgs: Input
executorOptions: object
Submits a Job to be executed by an executor, optionally passing in a JSON job argument your job handler function receives, and executor-specific submit options.
JavaScript
TypeScript
someAction.js
const submittedJob = await mySpecialJob.submit({ job: "args" })
delay(startAfter)
startAfter: int | string | Date required
Delaying the invocation of the job handler. The delay can be one of:
Integer: number of seconds to delay. [Default 0]
String: ISO date string to run at.
Date: Date to run at.
JavaScript
TypeScript
someAction.js
const submittedJob = await mySpecialJob
.delay(10)
.submit({ job: "args" }, { "retryLimit": 2 })
Tracking
The return value of submit() is an instance of SubmittedJob, which has the following fields:
jobId: The ID for the job in that executor.
jobName: The name of the job you used in your .wasp file.
executorName: The Symbol of the name of the job executor.
For pg-boss, you can import a Symbol from: import { PG_BOSS_EXECUTOR_NAME } from '@wasp/jobs/core/pgBoss/pgBossJob.js' if you wish to compare against executorName.
There are also some namespaced, job executor-specific objects.
For pg-boss, you may access: pgBoss
details(): pg-boss specific job detail information. Reference
cancel(): attempts to cancel a job. Reference
resume(): attempts to resume a canceled job. Reference | https://wasp-lang.dev/docs/0.11.8/advanced/jobs |
|
2 | null | 2024-04-23T15:15:28.364Z | https://wasp-lang.dev/docs/0.11.8/advanced/apis | https://wasp-lang.dev/docs/0.11.8 | http | ## Custom HTTP API Endpoints
In Wasp, the default client-server interaction mechanism is through [Operations](https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview). However, if you need a specific URL method/path, or a specific response, Operations may not be suitable for you. For these cases, you can use an `api`. Best of all, they should look and feel very familiar.
## How to Create an API[](#how-to-create-an-api "Direct link to How to Create an API")
APIs are used to tie a JS function to a certain endpoint e.g. `POST /something/special`. They are distinct from Operations and have no client-side helpers (like `useQuery`).
To create a Wasp API, you must:
1. Declare the API in Wasp using the `api` declaration
2. Define the API's NodeJS implementation
After completing these two steps, you'll be able to call the API from the client code (via our `Axios` wrapper), or from the outside world.
### Declaring the API in Wasp[](#declaring-the-api-in-wasp "Direct link to Declaring the API in Wasp")
First, we need to declare the API in the Wasp file and you can easily do this with the `api` declaration:
* JavaScript
* TypeScript
main.wasp
```
// ...api fooBar { // APIs and their implementations don't need to (but can) have the same name. fn: import { fooBar } from "@server/apis.js", httpRoute: (GET, "/foo/bar")}
```
Read more about the supported fields in the [API Reference](#api-reference).
### Defining the API's NodeJS Implementation[](#defining-the-apis-nodejs-implementation "Direct link to Defining the API's NodeJS Implementation")
After you defined the API, it should be implemented as a NodeJS function that takes three arguments:
1. `req`: Express Request object
2. `res`: Express Response object
3. `context`: An additional context object **injected into the API by Wasp**. This object contains user session information, as well as information about entities. The examples here won't use the context for simplicity purposes. You can read more about it in the [section about using entities in APIs](#using-entities-in-apis).
* JavaScript
* TypeScript
src/server/apis.js
```
export const fooBar = (req, res, context) => { res.set("Access-Control-Allow-Origin", "*"); // Example of modifying headers to override Wasp default CORS middleware. res.json({ msg: `Hello, ${context.user?.username || "stranger"}!` });};
```
## Using the API[](#using-the-api "Direct link to Using the API")
### Using the API externally[](#using-the-api-externally "Direct link to Using the API externally")
To use the API externally, you simply call the endpoint using the method and path you used.
For example, if your app is running at `https://example.com` then from the above you could issue a `GET` to `https://example/com/foo/callback` (in your browser, Postman, `curl`, another web service, etc.).
### Using the API from the Client[](#using-the-api-from-the-client "Direct link to Using the API from the Client")
To use the API from your client, including with auth support, you can import the Axios wrapper from `@wasp/api` and invoke a call. For example:
* JavaScript
* TypeScript
src/client/pages/SomePage.jsx
```
import React, { useEffect } from "react";import api from "@wasp/api";async function fetchCustomRoute() { const res = await api.get("/foo/bar"); console.log(res.data);}export const Foo = () => { useEffect(() => { fetchCustomRoute(); }, []); return <>// ...</>;};
```
#### Making Sure CORS Works[](#making-sure-cors-works "Direct link to Making Sure CORS Works")
APIs are designed to be as flexible as possible, hence they don't utilize the default middleware like Operations do. As a result, to use these APIs on the client side, you must ensure that CORS (Cross-Origin Resource Sharing) is enabled.
You can do this by defining custom middleware for your APIs in the Wasp file.
* JavaScript
* TypeScript
For example, an `apiNamespace` is a simple declaration used to apply some `middlewareConfigFn` to all APIs under some specific path:
main.wasp
```
apiNamespace fooBar { middlewareConfigFn: import { fooBarNamespaceMiddlewareFn } from "@server/apis.js", path: "/foo"}
```
And then in the implementation file:
src/server/apis.js
```
export const apiMiddleware = (config) => { return config;};
```
We are returning the default middleware which enables CORS for all APIs under the `/foo` path.
For more information about middleware configuration, please see: [Middleware Configuration](https://wasp-lang.dev/docs/0.11.8/advanced/middleware-config)
## Using Entities in APIs[](#using-entities-in-apis "Direct link to Using Entities in APIs")
In many cases, resources used in APIs will be [Entities](https://wasp-lang.dev/docs/0.11.8/data-model/entities). To use an Entity in your API, add it to the `api` declaration in Wasp:
* JavaScript
* TypeScript
main.wasp
```
api fooBar { fn: import { fooBar } from "@server/apis.js", entities: [Task], httpRoute: (GET, "/foo/bar")}
```
Wasp will inject the specified Entity into the APIs `context` argument, giving you access to the Entity's Prisma API:
* JavaScript
* TypeScript
src/server/apis.js
```
export const fooBar = (req, res, context) => { res.json({ count: await context.entities.Task.count() });};
```
The object `context.entities.Task` exposes `prisma.task` from [Prisma's CRUD API](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-client/crud).
## API Reference[](#api-reference "Direct link to API Reference")
* JavaScript
* TypeScript
main.wasp
```
api fooBar { fn: import { fooBar } from "@server/apis.js", httpRoute: (GET, "/foo/bar"), entities: [Task], auth: true, middlewareConfigFn: import { apiMiddleware } from "@server/apis.js"}
```
The `api` declaration has the following fields:
* `fn: ServerImport` required
The import statement of the APIs NodeJs implementation.
* `httpRoute: (HttpMethod, string)` required
The HTTP (method, path) pair, where the method can be one of:
* `ALL`, `GET`, `POST`, `PUT` or `DELETE`
* and path is an Express path `string`.
* `entities: [Entity]`
A list of entities you wish to use inside your API. You can read more about it [here](#using-entities-in-apis).
* `auth: bool`
If auth is enabled, this will default to `true` and provide a `context.user` object. If you do not wish to attempt to parse the JWT in the Authorization Header, you should set this to `false`.
* `middlewareConfigFn: ServerImport`
The import statement to an Express middleware config function for this API. See more in [middleware section](https://wasp-lang.dev/docs/0.11.8/advanced/middleware-config) of the docs. | null | https://wasp-lang.dev/docs/0.11.8/advanced/apis | In Wasp, the default client-server interaction mechanism is through Operations. However, if you need a specific URL method/path, or a specific response, Operations may not be suitable for you. For these cases, you can use an api. Best of all, they should look and feel very familiar. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf25f8738f23-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:28 GMT | Tue, 23 Apr 2024 15:25:28 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=Tbe38u%2Bs0H%2Fqn8bG%2FBIDYcoEWrgH3bcw7OHYS4tPf65p3KkMpLNmNUQNqHhoRVgSfKMsJtrbLWWWAencF9wh8it8ZhvtevLDBz45guTLZpSNs%2FP97YezQ5oSQehzgxb2"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | f4d9543621bc0413254a3f5cbacbfec3d061321f | null | 230E:3A1E9D:3CD311B:47D972D:6627D08F | HIT | MISS | cache-iad-kiad7000029-IAD | S1713885328.326482,VS0,VE13 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/advanced/apis | og:url | Custom HTTP API Endpoints | Wasp | og:title | In Wasp, the default client-server interaction mechanism is through Operations. However, if you need a specific URL method/path, or a specific response, Operations may not be suitable for you. For these cases, you can use an api. Best of all, they should look and feel very familiar. | og:description | Custom HTTP API Endpoints | Wasp | null | Custom HTTP API Endpoints
In Wasp, the default client-server interaction mechanism is through Operations. However, if you need a specific URL method/path, or a specific response, Operations may not be suitable for you. For these cases, you can use an api. Best of all, they should look and feel very familiar.
How to Create an API
APIs are used to tie a JS function to a certain endpoint e.g. POST /something/special. They are distinct from Operations and have no client-side helpers (like useQuery).
To create a Wasp API, you must:
Declare the API in Wasp using the api declaration
Define the API's NodeJS implementation
After completing these two steps, you'll be able to call the API from the client code (via our Axios wrapper), or from the outside world.
Declaring the API in Wasp
First, we need to declare the API in the Wasp file and you can easily do this with the api declaration:
JavaScript
TypeScript
main.wasp
// ...
api fooBar { // APIs and their implementations don't need to (but can) have the same name.
fn: import { fooBar } from "@server/apis.js",
httpRoute: (GET, "/foo/bar")
}
Read more about the supported fields in the API Reference.
Defining the API's NodeJS Implementation
After you defined the API, it should be implemented as a NodeJS function that takes three arguments:
req: Express Request object
res: Express Response object
context: An additional context object injected into the API by Wasp. This object contains user session information, as well as information about entities. The examples here won't use the context for simplicity purposes. You can read more about it in the section about using entities in APIs.
JavaScript
TypeScript
src/server/apis.js
export const fooBar = (req, res, context) => {
res.set("Access-Control-Allow-Origin", "*"); // Example of modifying headers to override Wasp default CORS middleware.
res.json({ msg: `Hello, ${context.user?.username || "stranger"}!` });
};
Using the API
Using the API externally
To use the API externally, you simply call the endpoint using the method and path you used.
For example, if your app is running at https://example.com then from the above you could issue a GET to https://example/com/foo/callback (in your browser, Postman, curl, another web service, etc.).
Using the API from the Client
To use the API from your client, including with auth support, you can import the Axios wrapper from @wasp/api and invoke a call. For example:
JavaScript
TypeScript
src/client/pages/SomePage.jsx
import React, { useEffect } from "react";
import api from "@wasp/api";
async function fetchCustomRoute() {
const res = await api.get("/foo/bar");
console.log(res.data);
}
export const Foo = () => {
useEffect(() => {
fetchCustomRoute();
}, []);
return <>// ...</>;
};
Making Sure CORS Works
APIs are designed to be as flexible as possible, hence they don't utilize the default middleware like Operations do. As a result, to use these APIs on the client side, you must ensure that CORS (Cross-Origin Resource Sharing) is enabled.
You can do this by defining custom middleware for your APIs in the Wasp file.
JavaScript
TypeScript
For example, an apiNamespace is a simple declaration used to apply some middlewareConfigFn to all APIs under some specific path:
main.wasp
apiNamespace fooBar {
middlewareConfigFn: import { fooBarNamespaceMiddlewareFn } from "@server/apis.js",
path: "/foo"
}
And then in the implementation file:
src/server/apis.js
export const apiMiddleware = (config) => {
return config;
};
We are returning the default middleware which enables CORS for all APIs under the /foo path.
For more information about middleware configuration, please see: Middleware Configuration
Using Entities in APIs
In many cases, resources used in APIs will be Entities. To use an Entity in your API, add it to the api declaration in Wasp:
JavaScript
TypeScript
main.wasp
api fooBar {
fn: import { fooBar } from "@server/apis.js",
entities: [Task],
httpRoute: (GET, "/foo/bar")
}
Wasp will inject the specified Entity into the APIs context argument, giving you access to the Entity's Prisma API:
JavaScript
TypeScript
src/server/apis.js
export const fooBar = (req, res, context) => {
res.json({ count: await context.entities.Task.count() });
};
The object context.entities.Task exposes prisma.task from Prisma's CRUD API.
API Reference
JavaScript
TypeScript
main.wasp
api fooBar {
fn: import { fooBar } from "@server/apis.js",
httpRoute: (GET, "/foo/bar"),
entities: [Task],
auth: true,
middlewareConfigFn: import { apiMiddleware } from "@server/apis.js"
}
The api declaration has the following fields:
fn: ServerImport required
The import statement of the APIs NodeJs implementation.
httpRoute: (HttpMethod, string) required
The HTTP (method, path) pair, where the method can be one of:
ALL, GET, POST, PUT or DELETE
and path is an Express path string.
entities: [Entity]
A list of entities you wish to use inside your API. You can read more about it here.
auth: bool
If auth is enabled, this will default to true and provide a context.user object. If you do not wish to attempt to parse the JWT in the Authorization Header, you should set this to false.
middlewareConfigFn: ServerImport
The import statement to an Express middleware config function for this API. See more in middleware section of the docs. | https://wasp-lang.dev/docs/0.11.8/advanced/apis |
|
2 | null | 2024-04-23T15:15:30.386Z | https://wasp-lang.dev/docs/0.11.8/advanced/middleware-config | https://wasp-lang.dev/docs/0.11.8 | http | Version: 0.11.8
## Configuring Middleware
Wasp comes with a minimal set of useful Express middleware in every application. While this is good for most users, we realize some may wish to add, modify, or remove some of these choices both globally, or on a per-`api`/path basis.
## Default Global Middleware 🌍[](#default-global-middleware- "Direct link to Default Global Middleware 🌍")
Wasp's Express server has the following middleware by default:
* [Helmet](https://helmetjs.github.io/): Helmet helps you secure your Express apps by setting various HTTP headers. _It's not a silver bullet, but it's a good start._
* [CORS](https://github.com/expressjs/cors#readme): CORS is a package for providing a middleware that can be used to enable [CORS](https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS) with various options.
note
CORS middleware is required for the frontend to communicate with the backend.
* [Morgan](https://github.com/expressjs/morgan#readme): HTTP request logger middleware.
* [express.json](https://expressjs.com/en/api.html#express.json) (which uses [body-parser](https://github.com/expressjs/body-parser#bodyparserjsonoptions)): parses incoming request bodies in a middleware before your handlers, making the result available under the `req.body` property.
note
JSON middlware is required for [Operations](https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview) to function properly.
* [express.urlencoded](https://expressjs.com/en/api.html#express.urlencoded) (which uses [body-parser](https://expressjs.com/en/resources/middleware/body-parser.html#bodyparserurlencodedoptions)): returns middleware that only parses urlencoded bodies and only looks at requests where the `Content-Type` header matches the type option.
* [cookieParser](https://github.com/expressjs/cookie-parser#readme): parses Cookie header and populates `req.cookies` with an object keyed by the cookie names.
## Customization[](#customization "Direct link to Customization")
You have three places where you can customize middleware:
1. [global](#1-customize-global-middleware): here, any changes will apply by default _to all operations (`query` and `action`) and `api`._ This is helpful if you wanted to add support for multiple domains to CORS, for example.
Modifying global middleware
Please treat modifications to global middleware with extreme care as they will affect all operations and APIs. If you are unsure, use one of the other two options.
2. [per-api](#2-customize-api-specific-middleware): you can override middleware for a specific api route (e.g. `POST /webhook/callback`). This is helpful if you want to disable JSON parsing for some callback, for example.
3. [per-path](#3-customize-per-path-middleware): this is helpful if you need to customize middleware for all methods under a given path.
* It's helpful for things like "complex CORS requests" which may need to apply to both `OPTIONS` and `GET`, or to apply some middleware to a _set of `api` routes_.
### Default Middleware Definitions[](#default-middleware-definitions "Direct link to Default Middleware Definitions")
Below is the actual definitions of default middleware which you can override.
* JavaScript
* TypeScript
```
const defaultGlobalMiddleware = new Map([ ['helmet', helmet()], ['cors', cors({ origin: config.allowedCORSOrigins })], ['logger', logger('dev')], ['express.json', express.json()], ['express.urlencoded', express.urlencoded({ extended: false })], ['cookieParser', cookieParser()]])
```
## 1\. Customize Global Middleware[](#1-customize-global-middleware "Direct link to 1. Customize Global Middleware")
If you would like to modify the middleware for _all_ operations and APIs, you can do something like:
* JavaScript
* TypeScript
main.wasp
```
app todoApp { // ... server: { setupFn: import setup from "@server/serverSetup.js", middlewareConfigFn: import { serverMiddlewareFn } from "@server/serverSetup.js" },}
```
src/server/serverSetup.js
```
import cors from 'cors'import config from '@wasp/config.js'export const serverMiddlewareFn = (middlewareConfig) => { // Example of adding extra domains to CORS. middlewareConfig.set('cors', cors({ origin: [config.frontendUrl, 'https://example1.com', 'https://example2.com'] })) return middlewareConfig}
```
## 2\. Customize `api`\-specific Middleware[](#2-customize-api-specific-middleware "Direct link to 2-customize-api-specific-middleware")
If you would like to modify the middleware for a single API, you can do something like:
* JavaScript
* TypeScript
main.wasp
```
// ...api webhookCallback { fn: import { webhookCallback } from "@server/apis.js", middlewareConfigFn: import { webhookCallbackMiddlewareFn } from "@server/apis.js", httpRoute: (POST, "/webhook/callback"), auth: false}
```
src/server/apis.js
```
import express from 'express'export const webhookCallback = (req, res, _context) => { res.json({ msg: req.body.length })}export const webhookCallbackMiddlewareFn = (middlewareConfig) => { console.log('webhookCallbackMiddlewareFn: Swap express.json for express.raw') middlewareConfig.delete('express.json') middlewareConfig.set('express.raw', express.raw({ type: '*/*' })) return middlewareConfig}
```
note
This gets installed on a per-method basis. Behind the scenes, this results in code like:
```
router.post('/webhook/callback', webhookCallbackMiddleware, ...)
```
## 3\. Customize Per-Path Middleware[](#3-customize-per-path-middleware "Direct link to 3. Customize Per-Path Middleware")
If you would like to modify the middleware for all API routes under some common path, you can define a `middlewareConfigFn` on an `apiNamespace`:
* JavaScript
* TypeScript
main.wasp
```
// ...apiNamespace fooBar { middlewareConfigFn: import { fooBarNamespaceMiddlewareFn } from "@server/apis.js", path: "/foo/bar"}
```
src/server/apis.js
```
export const fooBarNamespaceMiddlewareFn = (middlewareConfig) => { const customMiddleware = (_req, _res, next) => { console.log('fooBarNamespaceMiddlewareFn: custom middleware') next() } middlewareConfig.set('custom.middleware', customMiddleware) return middlewareConfig}
```
note
This gets installed at the router level for the path. Behind the scenes, this results in something like:
```
router.use('/foo/bar', fooBarNamespaceMiddleware)
``` | null | https://wasp-lang.dev/docs/0.11.8/advanced/middleware-config | Wasp comes with a minimal set of useful Express middleware in every application. While this is good for most users, we realize some may wish to add, modify, or remove some of these choices both globally, or on a per-api/path basis. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf327da1241d-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:30 GMT | Tue, 23 Apr 2024 15:25:30 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=Yt%2BWnYuoiTLNeUEG4thX8NPKjyXQsC%2BMQpvPAvj%2BvbXI1u8bGkt5GClZJDV4Rem4bujPEw1TifSFApm9kjzetI2h3jh1QnETC4l%2Bw%2Fcz45t4Vshf3Z%2BQr%2FzFi8k21fCM"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | b97552be40e0aa01954068d1b50e48aed4832640 | null | 98D0:179F03:3CAD300:47B3388:6627D092 | HIT | MISS | cache-iad-kiad7000059-IAD | S1713885330.344154,VS0,VE16 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/advanced/middleware-config | og:url | Configuring Middleware | Wasp | og:title | Wasp comes with a minimal set of useful Express middleware in every application. While this is good for most users, we realize some may wish to add, modify, or remove some of these choices both globally, or on a per-api/path basis. | og:description | Configuring Middleware | Wasp | null | Version: 0.11.8
Configuring Middleware
Wasp comes with a minimal set of useful Express middleware in every application. While this is good for most users, we realize some may wish to add, modify, or remove some of these choices both globally, or on a per-api/path basis.
Default Global Middleware 🌍
Wasp's Express server has the following middleware by default:
Helmet: Helmet helps you secure your Express apps by setting various HTTP headers. It's not a silver bullet, but it's a good start.
CORS: CORS is a package for providing a middleware that can be used to enable CORS with various options.
note
CORS middleware is required for the frontend to communicate with the backend.
Morgan: HTTP request logger middleware.
express.json (which uses body-parser): parses incoming request bodies in a middleware before your handlers, making the result available under the req.body property.
note
JSON middlware is required for Operations to function properly.
express.urlencoded (which uses body-parser): returns middleware that only parses urlencoded bodies and only looks at requests where the Content-Type header matches the type option.
cookieParser: parses Cookie header and populates req.cookies with an object keyed by the cookie names.
Customization
You have three places where you can customize middleware:
global: here, any changes will apply by default to all operations (query and action) and api. This is helpful if you wanted to add support for multiple domains to CORS, for example.
Modifying global middleware
Please treat modifications to global middleware with extreme care as they will affect all operations and APIs. If you are unsure, use one of the other two options.
per-api: you can override middleware for a specific api route (e.g. POST /webhook/callback). This is helpful if you want to disable JSON parsing for some callback, for example.
per-path: this is helpful if you need to customize middleware for all methods under a given path.
It's helpful for things like "complex CORS requests" which may need to apply to both OPTIONS and GET, or to apply some middleware to a set of api routes.
Default Middleware Definitions
Below is the actual definitions of default middleware which you can override.
JavaScript
TypeScript
const defaultGlobalMiddleware = new Map([
['helmet', helmet()],
['cors', cors({ origin: config.allowedCORSOrigins })],
['logger', logger('dev')],
['express.json', express.json()],
['express.urlencoded', express.urlencoded({ extended: false })],
['cookieParser', cookieParser()]
])
1. Customize Global Middleware
If you would like to modify the middleware for all operations and APIs, you can do something like:
JavaScript
TypeScript
main.wasp
app todoApp {
// ...
server: {
setupFn: import setup from "@server/serverSetup.js",
middlewareConfigFn: import { serverMiddlewareFn } from "@server/serverSetup.js"
},
}
src/server/serverSetup.js
import cors from 'cors'
import config from '@wasp/config.js'
export const serverMiddlewareFn = (middlewareConfig) => {
// Example of adding extra domains to CORS.
middlewareConfig.set('cors', cors({ origin: [config.frontendUrl, 'https://example1.com', 'https://example2.com'] }))
return middlewareConfig
}
2. Customize api-specific Middleware
If you would like to modify the middleware for a single API, you can do something like:
JavaScript
TypeScript
main.wasp
// ...
api webhookCallback {
fn: import { webhookCallback } from "@server/apis.js",
middlewareConfigFn: import { webhookCallbackMiddlewareFn } from "@server/apis.js",
httpRoute: (POST, "/webhook/callback"),
auth: false
}
src/server/apis.js
import express from 'express'
export const webhookCallback = (req, res, _context) => {
res.json({ msg: req.body.length })
}
export const webhookCallbackMiddlewareFn = (middlewareConfig) => {
console.log('webhookCallbackMiddlewareFn: Swap express.json for express.raw')
middlewareConfig.delete('express.json')
middlewareConfig.set('express.raw', express.raw({ type: '*/*' }))
return middlewareConfig
}
note
This gets installed on a per-method basis. Behind the scenes, this results in code like:
router.post('/webhook/callback', webhookCallbackMiddleware, ...)
3. Customize Per-Path Middleware
If you would like to modify the middleware for all API routes under some common path, you can define a middlewareConfigFn on an apiNamespace:
JavaScript
TypeScript
main.wasp
// ...
apiNamespace fooBar {
middlewareConfigFn: import { fooBarNamespaceMiddlewareFn } from "@server/apis.js",
path: "/foo/bar"
}
src/server/apis.js
export const fooBarNamespaceMiddlewareFn = (middlewareConfig) => {
const customMiddleware = (_req, _res, next) => {
console.log('fooBarNamespaceMiddlewareFn: custom middleware')
next()
}
middlewareConfig.set('custom.middleware', customMiddleware)
return middlewareConfig
}
note
This gets installed at the router level for the path. Behind the scenes, this results in something like:
router.use('/foo/bar', fooBarNamespaceMiddleware) | https://wasp-lang.dev/docs/0.11.8/advanced/middleware-config |
|
2 | null | 2024-04-23T15:15:30.918Z | https://wasp-lang.dev/docs/0.11.8/advanced/links | https://wasp-lang.dev/docs/0.11.8 | http | Version: 0.11.8
## Type-Safe Links
If you are using Typescript, you can use Wasp's custom `Link` component to create type-safe links to other pages on your site.
## Using the `Link` Component[](#using-the-link-component "Direct link to using-the-link-component")
After you defined a route:
main.wasp
```
route TaskRoute { path: "/task/:id", to: TaskPage }page TaskPage { ... }
```
You can get the benefits of type-safe links by using the `Link` component from `@wasp/router`:
TaskList.tsx
```
import { Link } from '@wasp/router'export const TaskList = () => { // ... return ( <div> {tasks.map((task) => ( <Link key={task.id} to="/task/:id" {/* 👆 You must provide a valid path here */} params={{ id: task.id }}> {/* 👆 All the params must be correctly passed in */} {task.description} </Link> ))} </div> )}
```
### Using Search Query & Hash[](#using-search-query--hash "Direct link to Using Search Query & Hash")
You can also pass `search` and `hash` props to the `Link` component:
TaskList.tsx
```
<Link to="/task/:id" params={{ id: task.id }} search={{ sortBy: 'date' }} hash="comments"> {task.description}</Link>
```
This will result in a link like this: `/task/1?sortBy=date#comments`. Check out the [API Reference](#link-component) for more details.
## The `routes` Object[](#the-routes-object "Direct link to the-routes-object")
You can also get all the pages in your app with the `routes` object:
TaskList.tsx
```
import { routes } from '@wasp/router'const linkToTask = routes.TaskRoute.build({ params: { id: 1 } })
```
This will result in a link like this: `/task/1`.
You can also pass `search` and `hash` props to the `build` function. Check out the [API Reference](#routes-object) for more details.
## API Reference[](#api-reference "Direct link to API Reference")
### `Link` Component[](#link-component "Direct link to link-component")
The `Link` component accepts the following props:
* `to` required
* A valid Wasp Route path from your `main.wasp` file.
* `params: { [name: string]: string | number }` required (if the path contains params)
* An object with keys and values for each param in the path.
* For example, if the path is `/task/:id`, then the `params` prop must be `{ id: 1 }`. Wasp supports required and optional params.
* `search: string[][] | Record<string, string> | string | URLSearchParams`
* Any valid input for `URLSearchParams` constructor.
* For example, the object `{ sortBy: 'date' }` becomes `?sortBy=date`.
* `hash: string`
* all other props that the `react-router-dom`'s [Link](https://v5.reactrouter.com/web/api/Link) component accepts
### `routes` Object[](#routes-object "Direct link to routes-object")
The `routes` object contains a function for each route in your app.
router.tsx
```
export const routes = { // RootRoute has a path like "/" RootRoute: { build: (options?: { search?: string[][] | Record<string, string> | string | URLSearchParams hash?: string }) => // ... }, // DetailRoute has a path like "/task/:id/:something?" DetailRoute: { build: ( options: { params: { id: ParamValue; something?: ParamValue; }, search?: string[][] | Record<string, string> | string | URLSearchParams hash?: string } ) => // ... }}
```
The `params` object is required if the route contains params. The `search` and `hash` parameters are optional.
You can use the `routes` object like this:
```
import { routes } from '@wasp/router'const linkToRoot = routes.RootRoute.build()const linkToTask = routes.DetailRoute.build({ params: { id: 1 } })
``` | null | https://wasp-lang.dev/docs/0.11.8/advanced/links | If you are using Typescript, you can use Wasp's custom Link component to create type-safe links to other pages on your site. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf33efca56fe-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:30 GMT | Tue, 23 Apr 2024 15:25:30 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=7MgmV8hsxILM0XmbDL5VQGeW2dLKN%2BEGX943L%2Frk3UZDbI5dmeWn%2FN%2BUdFvpZKm1GldhsTQlGjaEBZKHrjnJQLDKeePANsCC2qubRbewpoM%2FnF%2F2zFr7SXJlAJdap%2BHL"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 74503627039e4beeead455eb9b92537593e812d7 | null | ED7C:EB4F4:3BEA6BA:46F0842:6627D092 | HIT | MISS | cache-iad-kiad7000087-IAD | S1713885331.555564,VS0,VE12 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/advanced/links | og:url | Type-Safe Links | Wasp | og:title | If you are using Typescript, you can use Wasp's custom Link component to create type-safe links to other pages on your site. | og:description | Type-Safe Links | Wasp | null | Version: 0.11.8
Type-Safe Links
If you are using Typescript, you can use Wasp's custom Link component to create type-safe links to other pages on your site.
Using the Link Component
After you defined a route:
main.wasp
route TaskRoute { path: "/task/:id", to: TaskPage }
page TaskPage { ... }
You can get the benefits of type-safe links by using the Link component from @wasp/router:
TaskList.tsx
import { Link } from '@wasp/router'
export const TaskList = () => {
// ...
return (
<div>
{tasks.map((task) => (
<Link
key={task.id}
to="/task/:id"
{/* 👆 You must provide a valid path here */}
params={{ id: task.id }}>
{/* 👆 All the params must be correctly passed in */}
{task.description}
</Link>
))}
</div>
)
}
Using Search Query & Hash
You can also pass search and hash props to the Link component:
TaskList.tsx
<Link
to="/task/:id"
params={{ id: task.id }}
search={{ sortBy: 'date' }}
hash="comments"
>
{task.description}
</Link>
This will result in a link like this: /task/1?sortBy=date#comments. Check out the API Reference for more details.
The routes Object
You can also get all the pages in your app with the routes object:
TaskList.tsx
import { routes } from '@wasp/router'
const linkToTask = routes.TaskRoute.build({ params: { id: 1 } })
This will result in a link like this: /task/1.
You can also pass search and hash props to the build function. Check out the API Reference for more details.
API Reference
Link Component
The Link component accepts the following props:
to required
A valid Wasp Route path from your main.wasp file.
params: { [name: string]: string | number } required (if the path contains params)
An object with keys and values for each param in the path.
For example, if the path is /task/:id, then the params prop must be { id: 1 }. Wasp supports required and optional params.
search: string[][] | Record<string, string> | string | URLSearchParams
Any valid input for URLSearchParams constructor.
For example, the object { sortBy: 'date' } becomes ?sortBy=date.
hash: string
all other props that the react-router-dom's Link component accepts
routes Object
The routes object contains a function for each route in your app.
router.tsx
export const routes = {
// RootRoute has a path like "/"
RootRoute: {
build: (options?: {
search?: string[][] | Record<string, string> | string | URLSearchParams
hash?: string
}) => // ...
},
// DetailRoute has a path like "/task/:id/:something?"
DetailRoute: {
build: (
options: {
params: { id: ParamValue; something?: ParamValue; },
search?: string[][] | Record<string, string> | string | URLSearchParams
hash?: string
}
) => // ...
}
}
The params object is required if the route contains params. The search and hash parameters are optional.
You can use the routes object like this:
import { routes } from '@wasp/router'
const linkToRoot = routes.RootRoute.build()
const linkToTask = routes.DetailRoute.build({ params: { id: 1 } }) | https://wasp-lang.dev/docs/0.11.8/advanced/links |
|
2 | null | 2024-04-23T15:15:32.530Z | https://wasp-lang.dev/docs/0.11.8/general/cli | https://wasp-lang.dev/docs/0.11.8 | http | ## CLI Reference
This guide provides an overview of the Wasp CLI commands, arguments, and options.
## Overview[](#overview "Direct link to Overview")
Once [installed](https://wasp-lang.dev/docs/0.11.8/quick-start), you can use the wasp command from your command line.
If you run the `wasp` command without any arguments, it will show you a list of available commands and their descriptions:
```
USAGE wasp <command> [command-args]COMMANDS GENERAL new [<name>] [args] Creates a new Wasp project. Run it without arguments for interactive mode. OPTIONS: -t|--template <template-name> Check out the templates list here: https://github.com/wasp-lang/starters version Prints current version of CLI. waspls Run Wasp Language Server. Add --help to get more info. completion Prints help on bash completion. uninstall Removes Wasp from your system. IN PROJECT start Runs Wasp app in development mode, watching for file changes. start db Starts managed development database for you. db <db-cmd> [args] Executes a database command. Run 'wasp db' for more info. clean Deletes all generated code and other cached artifacts. Wasp equivalent of 'have you tried closing and opening it again?'. build Generates full web app code, ready for deployment. Use when deploying or ejecting. deploy Deploys your Wasp app to cloud hosting providers. telemetry Prints telemetry status. deps Prints the dependencies that Wasp uses in your project. dockerfile Prints the contents of the Wasp generated Dockerfile. info Prints basic information about current Wasp project. test Executes tests in your project.EXAMPLES wasp new MyApp wasp start wasp db migrate-devDocs: https://wasp-lang.dev/docsDiscord (chat): https://discord.gg/rzdnErXNewsletter: https://wasp-lang.dev/#signup
```
## Commands[](#commands "Direct link to Commands")
### Creating a New Project[](#creating-a-new-project "Direct link to Creating a New Project")
* Use `wasp new` to start the interactive mode for setting up a new Wasp project.
This will prompt you to input the project name and to select a template. The chosen template will then be used to generate the project directory with the specified name.
```
$ wasp new Enter the project name (e.g. my-project) ▸ MyFirstProject Choose a starter template [1] basic (default) [2] saas [3] todo-ts ▸ 1 🐝 --- Creating your project from the basic template... --------------------------- Created new Wasp app in ./MyFirstProject directory! To run it, do: cd MyFirstProject wasp start
```
* To skip the interactive mode and create a new Wasp project with the default template, use `wasp new <project-name>`.
```
$ wasp new MyFirstProject 🐝 --- Creating your project from the basic template... --------------------------- Created new Wasp app in ./MyFirstProject directory! To run it, do: cd MyFirstProject wasp start
```
### Project Commands[](#project-commands "Direct link to Project Commands")
* `wasp start` launches the Wasp app in development mode. It automatically opens a browser tab with your application running and watches for any changes to .wasp or files in `src/` to automatically reflect in the browser. It also shows messages from the web app, the server and the database on stdout/stderr.
* `wasp start db` starts the database for you. This can be very handy since you don't need to spin up your own database or provide its connection URL to the Wasp app.
* `wasp clean` removes all generated code and other cached artifacts. If using SQlite, it also deletes the SQlite database. Think of this as the Wasp version of the classic "turn it off and on again" solution.
```
$ wasp cleanDeleting .wasp/ directory...Deleted .wasp/ directory.
```
* `wasp build` generates the complete web app code, which is ready for [deployment](https://wasp-lang.dev/docs/0.11.8/advanced/deployment/overview). Use this command when you're deploying or ejecting. The generated code is stored in the `.wasp/build` folder.
* `wasp deploy` makes it easy to get your app hosted on the web.
Currently, Wasp offers support for [Fly.io](https://fly.io/). If you prefer a different hosting provider, feel free to let us know on Discord or submit a PR by updating [this TypeScript app](https://github.com/wasp-lang/wasp/tree/main/waspc/packages/deploy).
Read more about automatic deployment [here](https://wasp-lang.dev/docs/0.11.8/advanced/deployment/cli).
* `wasp telemetry` displays the status of [telemetry](https://wasp-lang.dev/docs/telemetry).
```
$ wasp telemetryTelemetry is currently: ENABLEDTelemetry cache directory: /home/user/.cache/wasp/telemetry/Last time telemetry data was sent for this project: 2021-05-27 09:21:16.79537226 UTCOur telemetry is anonymized and very limited in its scope: check https://wasp-lang.dev/docs/telemetry for more details.
```
* `wasp deps` lists the dependencies that Wasp uses in your project.
* `wasp info` provides basic details about the current Wasp project.
### Database Commands[](#database-commands "Direct link to Database Commands")
Wasp provides a suite of commands for managing the database. These commands all begin with `db` and primarily execute Prisma commands behind the scenes.
* `wasp db migrate-dev` synchronizes the development database with the current state of the schema (entities). If there are any changes in the schema, it generates a new migration and applies any pending migrations to the database.
* The `--name foo` option allows you to specify a name for the migration, while the `--create-only` option lets you create an empty migration without applying it.
* `wasp db studio` opens the GUI for inspecting your database.
### Bash Completion[](#bash-completion "Direct link to Bash Completion")
To set up Bash completion, run the `wasp completion` command and follow the instructions.
### Miscellaneous Commands[](#miscellaneous-commands "Direct link to Miscellaneous Commands")
* `wasp version` displays the current version of the CLI.
* `wasp uninstall` removes Wasp from your system.
```
$ wasp uninstall🐝 --- Uninstalling Wasp ... ------------------------------------------------------ We will remove the following directories: {home}/.local/share/wasp-lang/ {home}/.cache/wasp/ We will also remove the following files: {home}/.local/bin/wasp Are you sure you want to continue? [y/N] y ✅ --- Uninstalled Wasp -----------------------------------------------------------
``` | null | https://wasp-lang.dev/docs/0.11.8/general/cli | This guide provides an overview of the Wasp CLI commands, arguments, and options. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf3fd93c3955-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:32 GMT | Tue, 23 Apr 2024 15:25:32 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=BxF9uJpxTRFaq%2FOGFcSyny1pVvW%2Bv54srYijec0EVkm3j2Do9qHQbysn3FiMlLQGeb%2F21oV55cu8zC%2FbTyeDRHAXOi0cU1JP0oplmaLyO19taIZXNfsb3pfavYJPV4ve"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | b5e68afaf990e2597ed280a3a540c45cbde5d289 | null | 2E15:22319D:3A32D1D:4538A0C:6627D093 | HIT | MISS | cache-iad-kiad7000040-IAD | S1713885332.497051,VS0,VE11 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/general/cli | og:url | CLI Reference | Wasp | og:title | This guide provides an overview of the Wasp CLI commands, arguments, and options. | og:description | CLI Reference | Wasp | null | CLI Reference
This guide provides an overview of the Wasp CLI commands, arguments, and options.
Overview
Once installed, you can use the wasp command from your command line.
If you run the wasp command without any arguments, it will show you a list of available commands and their descriptions:
USAGE
wasp <command> [command-args]
COMMANDS
GENERAL
new [<name>] [args] Creates a new Wasp project. Run it without arguments for interactive mode.
OPTIONS:
-t|--template <template-name>
Check out the templates list here: https://github.com/wasp-lang/starters
version Prints current version of CLI.
waspls Run Wasp Language Server. Add --help to get more info.
completion Prints help on bash completion.
uninstall Removes Wasp from your system.
IN PROJECT
start Runs Wasp app in development mode, watching for file changes.
start db Starts managed development database for you.
db <db-cmd> [args] Executes a database command. Run 'wasp db' for more info.
clean Deletes all generated code and other cached artifacts.
Wasp equivalent of 'have you tried closing and opening it again?'.
build Generates full web app code, ready for deployment. Use when deploying or ejecting.
deploy Deploys your Wasp app to cloud hosting providers.
telemetry Prints telemetry status.
deps Prints the dependencies that Wasp uses in your project.
dockerfile Prints the contents of the Wasp generated Dockerfile.
info Prints basic information about current Wasp project.
test Executes tests in your project.
EXAMPLES
wasp new MyApp
wasp start
wasp db migrate-dev
Docs: https://wasp-lang.dev/docs
Discord (chat): https://discord.gg/rzdnErX
Newsletter: https://wasp-lang.dev/#signup
Commands
Creating a New Project
Use wasp new to start the interactive mode for setting up a new Wasp project.
This will prompt you to input the project name and to select a template. The chosen template will then be used to generate the project directory with the specified name.
$ wasp new
Enter the project name (e.g. my-project) ▸ MyFirstProject
Choose a starter template
[1] basic (default)
[2] saas
[3] todo-ts
▸ 1
🐝 --- Creating your project from the basic template... ---------------------------
Created new Wasp app in ./MyFirstProject directory!
To run it, do:
cd MyFirstProject
wasp start
To skip the interactive mode and create a new Wasp project with the default template, use wasp new <project-name>.
$ wasp new MyFirstProject
🐝 --- Creating your project from the basic template... ---------------------------
Created new Wasp app in ./MyFirstProject directory!
To run it, do:
cd MyFirstProject
wasp start
Project Commands
wasp start launches the Wasp app in development mode. It automatically opens a browser tab with your application running and watches for any changes to .wasp or files in src/ to automatically reflect in the browser. It also shows messages from the web app, the server and the database on stdout/stderr.
wasp start db starts the database for you. This can be very handy since you don't need to spin up your own database or provide its connection URL to the Wasp app.
wasp clean removes all generated code and other cached artifacts. If using SQlite, it also deletes the SQlite database. Think of this as the Wasp version of the classic "turn it off and on again" solution.
$ wasp clean
Deleting .wasp/ directory...
Deleted .wasp/ directory.
wasp build generates the complete web app code, which is ready for deployment. Use this command when you're deploying or ejecting. The generated code is stored in the .wasp/build folder.
wasp deploy makes it easy to get your app hosted on the web.
Currently, Wasp offers support for Fly.io. If you prefer a different hosting provider, feel free to let us know on Discord or submit a PR by updating this TypeScript app.
Read more about automatic deployment here.
wasp telemetry displays the status of telemetry.
$ wasp telemetry
Telemetry is currently: ENABLED
Telemetry cache directory: /home/user/.cache/wasp/telemetry/
Last time telemetry data was sent for this project: 2021-05-27 09:21:16.79537226 UTC
Our telemetry is anonymized and very limited in its scope: check https://wasp-lang.dev/docs/telemetry for more details.
wasp deps lists the dependencies that Wasp uses in your project.
wasp info provides basic details about the current Wasp project.
Database Commands
Wasp provides a suite of commands for managing the database. These commands all begin with db and primarily execute Prisma commands behind the scenes.
wasp db migrate-dev synchronizes the development database with the current state of the schema (entities). If there are any changes in the schema, it generates a new migration and applies any pending migrations to the database.
The --name foo option allows you to specify a name for the migration, while the --create-only option lets you create an empty migration without applying it.
wasp db studio opens the GUI for inspecting your database.
Bash Completion
To set up Bash completion, run the wasp completion command and follow the instructions.
Miscellaneous Commands
wasp version displays the current version of the CLI.
wasp uninstall removes Wasp from your system.
$ wasp uninstall
🐝 --- Uninstalling Wasp ... ------------------------------------------------------
We will remove the following directories:
{home}/.local/share/wasp-lang/
{home}/.cache/wasp/
We will also remove the following files:
{home}/.local/bin/wasp
Are you sure you want to continue? [y/N]
y
✅ --- Uninstalled Wasp ----------------------------------------------------------- | https://wasp-lang.dev/docs/0.11.8/general/cli |
|
2 | null | 2024-04-23T15:15:32.515Z | https://wasp-lang.dev/docs/0.11.8/general/language | https://wasp-lang.dev/docs/0.11.8 | http | ## Wasp Language (.wasp)
Wasp language (what you write in .wasp files) is a declarative, statically typed, domain-specific language (DSL).
It is a quite simple language, closer to JSON, CSS or SQL than to e.g. Javascript or Python, since it is not a general programming language, but more of a configuration language.
It is pretty intuitive to learn (there isn't much to learn really!) and you can probably do just fine without reading this page and learning from the rest of the docs as you go, but if you want a bit more formal definition and deeper understanding of how it works, then read on!
## Declarations[](#declarations "Direct link to Declarations")
The central point of Wasp language are **declarations**, and Wasp code is at the end just a bunch of declarations, each of them describing a part of your web app.
```
app MyApp { title: "My app"}route RootRoute { path: "/", to: DashboardPage }page DashboardPage { component: import Dashboard from "@client/Dashboard.js"}
```
In the example above we described a web app via three declarations: `app MyApp { ... }`, `route RootRoute { ... }` and `page DashboardPage { ... }`.
Syntax for writing a declaration is `<declaration_type> <declaration_name> <declaration_body>`, where:
* `<declaration_type>` is one of the declaration types offered by Wasp (`app`, `route`, ...)
* `<declaration_name>` is an identifier chosen by you to name this specific declaration
* `<declaration_body>` is the value/definition of the declaration itself, which has to match the specific declaration body type expected by the chosen declaration type.
So, for `app` declaration above, we have:
* declaration type `app`
* declaration name `MyApp` (we could have used any other identifier, like `foobar`, `foo_bar`, or `hi3Ho`)
* declaration body `{ title: "My app" }`, which is a dictionary with field `title` that has string value. Type of this dictionary is in line with the declaration body type of the `app` declaration type. If we provided something else, e.g. changed `title` to `little`, we would get a type error from Wasp compiler since that does not match the expected type of the declaration body for `app`.
Each declaration has a meaning behind it that describes how your web app should behave and function.
All the other types in Wasp language (primitive types (`string`, `number`), composite types (`dict`, `list`), enum types (`DbSystem`), ...) are used to define the declaration bodies.
## Complete List of Wasp Types[](#complete-list-of-wasp-types "Direct link to Complete List of Wasp Types")
Wasp's type system can be divided into two main categories of types: **fundamental types** and **domain types**.
While fundamental types are here to be basic building blocks of a a language, and are very similar to what you would see in other popular languages, domain types are what makes Wasp special, as they model the concepts of a web app like `page`, `route` and similar.
* Fundamental types ([source of truth](https://github.com/wasp-lang/wasp/blob/main/waspc/src/Wasp/Analyzer/Type.hs))
* Primitive types
* **string** (`"foo"`, `"they said: \"hi\""`)
* **bool** (`true`, `false`)
* **number** (`12`, `14.5`)
* **declaration reference** (name of existing declaration: `TaskPage`, `updateTask`)
* **ServerImport** (external server import) (`import Foo from "@server/bar.js"`, `import { Smth } from "@server/a/b.js"`)
* The path has to start with "@server". The rest is relative to the `src/server` directory.
* import has to be a default import `import Foo` or a single named import `import { Foo }`.
* **ClientImport** (external client import) (`import Foo from "@client/bar.js"`, `import { Smth } from "@client/a/b.js"`)
* The path has to start with "@client". The rest is relative to the `src/client` directory.
* import has to be a default import `import Foo` or a single named import `import { Foo }`.
* **json** (`{=json { a: 5, b: ["hi"] } json=}`)
* **psl** (Prisma Schema Language) (`{=psl <psl data model syntax> psl=}`)
* Check [Prisma docs](https://www.prisma.io/docs/concepts/components/prisma-schema/data-model) for the syntax of psl data model.
* Composite types
* **dict** (dictionary) (`{ a: 5, b: "foo" }`)
* **list** (`[1, 2, 3]`)
* **tuple** (`(1, "bar")`, `(2, 4, true)`)
* Tuples can be of size 2, 3 and 4.
* Domain types ([source of truth](https://github.com/wasp-lang/wasp/blob/main/waspc/src/Wasp/Analyzer/StdTypeDefinitions.hs))
* Declaration types
* **action**
* **api**
* **apiNamespace**
* **app**
* **entity**
* **job**
* **page**
* **query**
* **route**
* **crud**
* Enum types
* **DbSystem**
* **HttpMethod**
* **JobExecutor**
* **EmailProvider**
You can find more details about each of the domain types, both regarding their body types and what they mean, in the corresponding doc pages covering their features. | null | https://wasp-lang.dev/docs/0.11.8/general/language | Wasp language (what you write in .wasp files) is a declarative, statically typed, domain-specific language (DSL). | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf3fff6f9c31-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:32 GMT | Tue, 23 Apr 2024 15:04:53 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=CiYHITdnCnJUIFCROQOQel70TZpjkxBtO%2FQwpu8ddegt0G26n87MaryNS9dpfzXcj9DAVvbTDlesFLjK3pQVyfbEVfhRutR1Exz8TFD4Z7M1Ex4e59qyiw%2FUXE%2BAODeL"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | HIT | 0 | e5a9ab7b8afdb94f6845b2d2e05a082bd22fc548 | null | 833E:169A:1E5F95:24403B:6627CBBD | null | MISS | cache-iad-kiad7000022-IAD | S1713885332.487648,VS0,VE12 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/general/language | og:url | Wasp Language (.wasp) | Wasp | og:title | Wasp language (what you write in .wasp files) is a declarative, statically typed, domain-specific language (DSL). | og:description | Wasp Language (.wasp) | Wasp | null | Wasp Language (.wasp)
Wasp language (what you write in .wasp files) is a declarative, statically typed, domain-specific language (DSL).
It is a quite simple language, closer to JSON, CSS or SQL than to e.g. Javascript or Python, since it is not a general programming language, but more of a configuration language.
It is pretty intuitive to learn (there isn't much to learn really!) and you can probably do just fine without reading this page and learning from the rest of the docs as you go, but if you want a bit more formal definition and deeper understanding of how it works, then read on!
Declarations
The central point of Wasp language are declarations, and Wasp code is at the end just a bunch of declarations, each of them describing a part of your web app.
app MyApp {
title: "My app"
}
route RootRoute { path: "/", to: DashboardPage }
page DashboardPage {
component: import Dashboard from "@client/Dashboard.js"
}
In the example above we described a web app via three declarations: app MyApp { ... }, route RootRoute { ... } and page DashboardPage { ... }.
Syntax for writing a declaration is <declaration_type> <declaration_name> <declaration_body>, where:
<declaration_type> is one of the declaration types offered by Wasp (app, route, ...)
<declaration_name> is an identifier chosen by you to name this specific declaration
<declaration_body> is the value/definition of the declaration itself, which has to match the specific declaration body type expected by the chosen declaration type.
So, for app declaration above, we have:
declaration type app
declaration name MyApp (we could have used any other identifier, like foobar, foo_bar, or hi3Ho)
declaration body { title: "My app" }, which is a dictionary with field title that has string value. Type of this dictionary is in line with the declaration body type of the app declaration type. If we provided something else, e.g. changed title to little, we would get a type error from Wasp compiler since that does not match the expected type of the declaration body for app.
Each declaration has a meaning behind it that describes how your web app should behave and function.
All the other types in Wasp language (primitive types (string, number), composite types (dict, list), enum types (DbSystem), ...) are used to define the declaration bodies.
Complete List of Wasp Types
Wasp's type system can be divided into two main categories of types: fundamental types and domain types.
While fundamental types are here to be basic building blocks of a a language, and are very similar to what you would see in other popular languages, domain types are what makes Wasp special, as they model the concepts of a web app like page, route and similar.
Fundamental types (source of truth)
Primitive types
string ("foo", "they said: \"hi\"")
bool (true, false)
number (12, 14.5)
declaration reference (name of existing declaration: TaskPage, updateTask)
ServerImport (external server import) (import Foo from "@server/bar.js", import { Smth } from "@server/a/b.js")
The path has to start with "@server". The rest is relative to the src/server directory.
import has to be a default import import Foo or a single named import import { Foo }.
ClientImport (external client import) (import Foo from "@client/bar.js", import { Smth } from "@client/a/b.js")
The path has to start with "@client". The rest is relative to the src/client directory.
import has to be a default import import Foo or a single named import import { Foo }.
json ({=json { a: 5, b: ["hi"] } json=})
psl (Prisma Schema Language) ({=psl <psl data model syntax> psl=})
Check Prisma docs for the syntax of psl data model.
Composite types
dict (dictionary) ({ a: 5, b: "foo" })
list ([1, 2, 3])
tuple ((1, "bar"), (2, 4, true))
Tuples can be of size 2, 3 and 4.
Domain types (source of truth)
Declaration types
action
api
apiNamespace
app
entity
job
page
query
route
crud
Enum types
DbSystem
HttpMethod
JobExecutor
EmailProvider
You can find more details about each of the domain types, both regarding their body types and what they mean, in the corresponding doc pages covering their features. | https://wasp-lang.dev/docs/0.11.8/general/language |
|
2 | null | 2024-04-23T15:15:33.333Z | https://wasp-lang.dev/docs/0.11.8/editor-setup | https://wasp-lang.dev/docs/0.11.8 | http | ## Editor Setup
note
This page assumes you have already installed Wasp. If you do not have Wasp installed yet, check out the [Quick Start](https://wasp-lang.dev/docs/0.11.8/quick-start) guide.
Wasp comes with the Wasp language server, which gives supported editors powerful support and integration with the language.
## VSCode[](#vscode "Direct link to VSCode")
Currently, Wasp only supports integration with VSCode. Install the [Wasp language extension](https://marketplace.visualstudio.com/items?itemName=wasp-lang.wasp) to get syntax highlighting and integration with the Wasp language server.
The extension enables:
* syntax highlighting for `.wasp` files
* scaffolding of new project files
* code completion
* diagnostics (errors and warnings)
* go to definition
and more! | null | https://wasp-lang.dev/docs/0.11.8/editor-setup | This page assumes you have already installed Wasp. If you do not have Wasp installed yet, check out the Quick Start guide. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf44fb4e59ce-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:33 GMT | Tue, 23 Apr 2024 15:25:33 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=d6dvKsaR7S4RcTErEdKUzXrz2bdnciRQSIEWqNAtill2aCr2RuRLrE4yDXELAIWohRe39EIXaSRVIb1e7%2Fg8OAU4uWCkEllxcExddpe1ZChgQVY8udlJ%2FOJB62wtCZy7"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | da09892f58ce881f9d8311abd0d749f864b19d20 | null | 3B92:EB4F4:3BEA8A1:46F0A7C:6627D093 | HIT | MISS | cache-iad-kiad7000160-IAD | S1713885333.300223,VS0,VE17 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/editor-setup | og:url | Editor Setup | Wasp | og:title | This page assumes you have already installed Wasp. If you do not have Wasp installed yet, check out the Quick Start guide. | og:description | Editor Setup | Wasp | null | Editor Setup
note
This page assumes you have already installed Wasp. If you do not have Wasp installed yet, check out the Quick Start guide.
Wasp comes with the Wasp language server, which gives supported editors powerful support and integration with the language.
VSCode
Currently, Wasp only supports integration with VSCode. Install the Wasp language extension to get syntax highlighting and integration with the Wasp language server.
The extension enables:
syntax highlighting for .wasp files
scaffolding of new project files
code completion
diagnostics (errors and warnings)
go to definition
and more! | https://wasp-lang.dev/docs/0.11.8/editor-setup |
|
2 | null | 2024-04-23T15:15:33.209Z | https://wasp-lang.dev/docs/0.11.8/quick-start | https://wasp-lang.dev/docs/0.11.8 | http | ## Quick Start
## Installation[](#installation "Direct link to Installation")
Try Wasp Without Installing 🤔?
Give Wasp a spin in the browser without any setup by running our [Wasp Template for Gitpod](https://github.com/wasp-lang/gitpod-template)
Welcome, new Waspeteer 🐝!
To install Wasp on Linux / OSX / WSL(Win), open your terminal and run:
```
curl -sSL https://get.wasp-lang.dev/installer.sh | sh
```
ℹ️ Wasp requires Node.js and will warn you if it is missing: check below for [more details](#requirements).
Then, create a new app by running:
and then run the app:
```
cd <my-project-name>wasp start
```
That's it 🎉 You have successfully created and served a new web app at [http://localhost:3000](http://localhost:3000/) and Wasp is serving both frontend and backend for you.
Something Unclear?
Check [More Details](#more-details) section below if anything went wrong, or if you have additional questions.
### What next?[](#what-next "Direct link to What next?")
* 👉 **Check out the [Todo App tutorial](https://wasp-lang.dev/docs/0.11.8/tutorial/create), which will take you through all the core features of Wasp!** 👈
* [Setup your editor](https://wasp-lang.dev/docs/0.11.8/editor-setup) for working with Wasp.
* Join us on [Discord](https://discord.gg/rzdnErX)! Any feedback or questions you have, we are there for you.
* Follow Wasp development by subscribing to our newsletter: [https://wasp-lang.dev/#signup](https://wasp-lang.dev/#signup) . We usually send 1 per month, and Matija does his best to unleash his creativity to make them engaging and fun to read :D!
* * *
## More details[](#more-details "Direct link to More details")
### Requirements[](#requirements "Direct link to Requirements")
You must have Node.js (and NPM) installed on your machine and available in `PATH`. We rely on the latest Node.js LTS version (currently `v18.14.2`).
We recommend using [nvm](https://github.com/nvm-sh/nvm) for managing your Node.js installation version(s).
Quick guide on installing/using nvm
Install nvm via your OS package manager (`apt`, `pacman`, `homebrew`, ...) or via the [nvm](https://github.com/nvm-sh/nvm#install--update-script) install script.
Then, install a version of Node.js that you need:
Finally, whenever you need to ensure a specific version of Node.js is used, run:
to set the Node.js version for the current shell session.
You can run
to check the version of Node.js currently being used in this shell session.
Check NVM repo for more details: [https://github.com/nvm-sh/nvm](https://github.com/nvm-sh/nvm).
### Installation[](#installation-1 "Direct link to Installation")
* Linux / macOS
* Windows
* From source
Open your terminal and run:
```
curl -sSL https://get.wasp-lang.dev/installer.sh | sh
```
Running Wasp on Mac with Mx chip (arm64)
**Experiencing the 'Bad CPU type in executable' issue on a device with arm64 (Apple Silicon)?** Given that the wasp binary is built for x86 and not for arm64 (Apple Silicon), you'll need to install [Rosetta on your Mac](https://support.apple.com/en-us/HT211861) if you are using a Mac with Mx (M1, M2, ...). Rosetta is a translation process that enables users to run applications designed for x86 on arm64 (Apple Silicon). To install Rosetta, run the following command in your terminal
```
softwareupdate --install-rosetta
```
Once Rosetta is installed, you should be able to run Wasp without any issues. | null | https://wasp-lang.dev/docs/0.11.8/quick-start | Installation | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf443de38197-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:33 GMT | Tue, 23 Apr 2024 15:25:33 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=ArtWgtPUCjYMymDlQ8p8RT7pPsGMeLvZWotrVc%2FIzzrBjfr%2FEERvyBmLcxzqIXj4%2BRIJpNItU3B2IRkjpv%2FMw9MAS%2B7lp%2FDK%2FAYyWSLDVokMqLROsWGw1ARkBLIveefP"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | f91734654a1ecf7834ba9e40414a49e798e41128 | null | A578:16D7:486EA3:55883D:6627D095 | HIT | MISS | cache-iad-kiad7000033-IAD | S1713885333.181111,VS0,VE11 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/quick-start | og:url | Quick Start | Wasp | og:title | Installation | og:description | Quick Start | Wasp | null | Quick Start
Installation
Try Wasp Without Installing 🤔?
Give Wasp a spin in the browser without any setup by running our Wasp Template for Gitpod
Welcome, new Waspeteer 🐝!
To install Wasp on Linux / OSX / WSL(Win), open your terminal and run:
curl -sSL https://get.wasp-lang.dev/installer.sh | sh
ℹ️ Wasp requires Node.js and will warn you if it is missing: check below for more details.
Then, create a new app by running:
and then run the app:
cd <my-project-name>
wasp start
That's it 🎉 You have successfully created and served a new web app at http://localhost:3000 and Wasp is serving both frontend and backend for you.
Something Unclear?
Check More Details section below if anything went wrong, or if you have additional questions.
What next?
👉 Check out the Todo App tutorial, which will take you through all the core features of Wasp! 👈
Setup your editor for working with Wasp.
Join us on Discord! Any feedback or questions you have, we are there for you.
Follow Wasp development by subscribing to our newsletter: https://wasp-lang.dev/#signup . We usually send 1 per month, and Matija does his best to unleash his creativity to make them engaging and fun to read :D!
More details
Requirements
You must have Node.js (and NPM) installed on your machine and available in PATH. We rely on the latest Node.js LTS version (currently v18.14.2).
We recommend using nvm for managing your Node.js installation version(s).
Quick guide on installing/using nvm
Install nvm via your OS package manager (apt, pacman, homebrew, ...) or via the nvm install script.
Then, install a version of Node.js that you need:
Finally, whenever you need to ensure a specific version of Node.js is used, run:
to set the Node.js version for the current shell session.
You can run
to check the version of Node.js currently being used in this shell session.
Check NVM repo for more details: https://github.com/nvm-sh/nvm.
Installation
Linux / macOS
Windows
From source
Open your terminal and run:
curl -sSL https://get.wasp-lang.dev/installer.sh | sh
Running Wasp on Mac with Mx chip (arm64)
Experiencing the 'Bad CPU type in executable' issue on a device with arm64 (Apple Silicon)? Given that the wasp binary is built for x86 and not for arm64 (Apple Silicon), you'll need to install Rosetta on your Mac if you are using a Mac with Mx (M1, M2, ...). Rosetta is a translation process that enables users to run applications designed for x86 on arm64 (Apple Silicon). To install Rosetta, run the following command in your terminal
softwareupdate --install-rosetta
Once Rosetta is installed, you should be able to run Wasp without any issues. | https://wasp-lang.dev/docs/0.11.8/quick-start |
|
2 | null | 2024-04-23T15:15:34.621Z | https://wasp-lang.dev/docs/0.11.8/tutorial/project-structure | https://wasp-lang.dev/docs/0.11.8 | http | ## 2\. Project Structure
After creating a new Wasp project, you'll get a file structure that looks like this:
```
.├── .gitignore├── main.wasp # Your Wasp code goes here.├── src│ ├── client # Your client code (JS/CSS/HTML) goes here.│ │ ├── Main.css│ │ ├── MainPage.jsx│ │ ├── tsconfig.json│ │ ├── vite.config.ts│ │ ├── vite-env.d.ts│ │ └── waspLogo.png│ ├── server # Your server code (Node JS) goes here.│ │ └── tsconfig.json│ ├── shared # Your shared (runtime independent) code goes here.│ │ └── tsconfig.json│ └── .waspignore└── .wasproot
```
By _your code_, we mean the _"the code you write"_, as opposed to the code generated by Wasp. Wasp expects you to separate all of your code—which we call external code—into three folders to make it obvious how each file is executed:
* `src/client`: Contains the code executed on the client, in the browser.
* `src/server`: Contains the code executed on the server, with Node.
* `src/shared`: Contains code that may be executed on both the client and server.
Many of the other files (`tsconfig.json`, `vite-env.d.ts`, etc.) are used by your IDE to improve your development experience with tools like autocompletion, intellisense, and error reporting. The file `vite.config.ts` is used to configure [Vite](https://vitejs.dev/guide/), Wasp's build tool of choice. We won't be configuring Vite in this tutorial, so you can safely ignore the file. Still, if you ever end up wanting more control over Vite, you'll find everything you need to know in [custom Vite config docs](https://wasp-lang.dev/docs/0.11.8/project/custom-vite-config).
TypeScript Support
Wasp supports TypeScript out of the box, but you are free to choose between or mix JavaScript and TypeScript as you see fit.
We'll provide you with both JavaScript and TypeScript code in this tutorial. Code blocks will have a toggle to switch between vanilla 🍦 JavaScript and TypeScript.
The most important file in the project is `main.wasp`. Wasp uses the configuration within it to perform its magic. Based on what you write, it generates a bunch of code for your database, server-client communication, React routing, and more.
Let's look a bit closer at `main.wasp.`
## `main.wasp`[](#mainwasp "Direct link to mainwasp")
This file, written in our Wasp configuration language, defines your app and lets Wasp take care a ton of features to your app for you. The file contains several _declarations_ which, together, describe all the components of your app.
The default Wasp file generated via `wasp new` on the previous page looks like:
* JavaScript
* TypeScript
main.wasp
```
app TodoApp { wasp: { version: "^0.11.6" // Pins the version of Wasp to use. }, title: "Todo app" // Used as the browser tab title. Note that all strings in Wasp are double quoted!}route RootRoute { path: "/", to: MainPage }page MainPage { // We specify that the React implementation of the page is the default export // of `src/client/MainPage.jsx`. This statement uses standard JS import syntax. // Use `@client` to reference files inside the `src/client` folder. component: import Main from "@client/MainPage.jsx"}
```
This file uses three declaration types:
* **app**: Top-level configuration information about your app.
* **route**: Describes which path each page should be accessible from.
* **page**: Defines a web page and the React component that will be rendered when the page is loaded.
In the next section, we'll explore how **route** and **page** work together to build your web app. | null | https://wasp-lang.dev/docs/0.11.8/tutorial/project-structure | After creating a new Wasp project, you'll get a file structure that looks like this: | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf4cb9dd593e-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:34 GMT | Tue, 23 Apr 2024 15:25:34 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=XvOFlgpAMBu7KH74p84G%2F1RFQFV2oR8EUEwSQdIoJdpwXbMOpipyfuUuhIDOjGckF3KxkpH0A4yrbyXK9d3JtZbVKAqmgC%2FvsCd%2BJ7VhvlmbDxZ5%2BBFFGEvyk%2F19kAAa"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 024e71b499071b822020a62cf4667810d4166cf6 | null | 708E:16D6:312F82:3AA6B8:6627D096 | null | MISS | cache-iad-kiad7000084-IAD | S1713885335.549522,VS0,VE32 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/tutorial/project-structure | og:url | 2. Project Structure | Wasp | og:title | After creating a new Wasp project, you'll get a file structure that looks like this: | og:description | 2. Project Structure | Wasp | null | 2. Project Structure
After creating a new Wasp project, you'll get a file structure that looks like this:
.
├── .gitignore
├── main.wasp # Your Wasp code goes here.
├── src
│ ├── client # Your client code (JS/CSS/HTML) goes here.
│ │ ├── Main.css
│ │ ├── MainPage.jsx
│ │ ├── tsconfig.json
│ │ ├── vite.config.ts
│ │ ├── vite-env.d.ts
│ │ └── waspLogo.png
│ ├── server # Your server code (Node JS) goes here.
│ │ └── tsconfig.json
│ ├── shared # Your shared (runtime independent) code goes here.
│ │ └── tsconfig.json
│ └── .waspignore
└── .wasproot
By your code, we mean the "the code you write", as opposed to the code generated by Wasp. Wasp expects you to separate all of your code—which we call external code—into three folders to make it obvious how each file is executed:
src/client: Contains the code executed on the client, in the browser.
src/server: Contains the code executed on the server, with Node.
src/shared: Contains code that may be executed on both the client and server.
Many of the other files (tsconfig.json, vite-env.d.ts, etc.) are used by your IDE to improve your development experience with tools like autocompletion, intellisense, and error reporting. The file vite.config.ts is used to configure Vite, Wasp's build tool of choice. We won't be configuring Vite in this tutorial, so you can safely ignore the file. Still, if you ever end up wanting more control over Vite, you'll find everything you need to know in custom Vite config docs.
TypeScript Support
Wasp supports TypeScript out of the box, but you are free to choose between or mix JavaScript and TypeScript as you see fit.
We'll provide you with both JavaScript and TypeScript code in this tutorial. Code blocks will have a toggle to switch between vanilla 🍦 JavaScript and TypeScript.
The most important file in the project is main.wasp. Wasp uses the configuration within it to perform its magic. Based on what you write, it generates a bunch of code for your database, server-client communication, React routing, and more.
Let's look a bit closer at main.wasp.
main.wasp
This file, written in our Wasp configuration language, defines your app and lets Wasp take care a ton of features to your app for you. The file contains several declarations which, together, describe all the components of your app.
The default Wasp file generated via wasp new on the previous page looks like:
JavaScript
TypeScript
main.wasp
app TodoApp {
wasp: {
version: "^0.11.6" // Pins the version of Wasp to use.
},
title: "Todo app" // Used as the browser tab title. Note that all strings in Wasp are double quoted!
}
route RootRoute { path: "/", to: MainPage }
page MainPage {
// We specify that the React implementation of the page is the default export
// of `src/client/MainPage.jsx`. This statement uses standard JS import syntax.
// Use `@client` to reference files inside the `src/client` folder.
component: import Main from "@client/MainPage.jsx"
}
This file uses three declaration types:
app: Top-level configuration information about your app.
route: Describes which path each page should be accessible from.
page: Defines a web page and the React component that will be rendered when the page is loaded.
In the next section, we'll explore how route and page work together to build your web app. | https://wasp-lang.dev/docs/0.11.8/tutorial/project-structure |
|
2 | null | 2024-04-23T15:15:35.542Z | https://wasp-lang.dev/docs/0.11.8/tutorial/entities | https://wasp-lang.dev/docs/0.11.8 | http | ```
// ...entity Task {=psl id Int @id @default(autoincrement()) description String isDone Boolean @default(false)psl=}
``` | null | https://wasp-lang.dev/docs/0.11.8/tutorial/entities | Entities are one of the most important concepts in Wasp and are how you define what gets stored in the database. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf527cfc3824-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:35 GMT | Tue, 23 Apr 2024 15:25:35 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=ahNE%2BhEH02Eaf6lI4S6fV0b20qOHQ2n%2B3oFFsHXKqNlePlW6oLs2XKgwyL0jAGoPCrI3PIsB2Iuz%2BC19euwFQkA%2FEelh%2By%2BZy4XIMWMgVZv6BX%2BlB9HKZymqBWzF6qW4"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 1fe01c9da2c8cf8878febd912d656a0f4ed33bc2 | null | FACE:32522B:39FC334:45025DC:6627D097 | HIT | MISS | cache-iad-kiad7000074-IAD | S1713885335.453052,VS0,VE10 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/tutorial/entities | og:url | 4. Database Entities | Wasp | og:title | Entities are one of the most important concepts in Wasp and are how you define what gets stored in the database. | og:description | 4. Database Entities | Wasp | null | // ...
entity Task {=psl
id Int @id @default(autoincrement())
description String
isDone Boolean @default(false)
psl=} | https://wasp-lang.dev/docs/0.11.8/tutorial/entities |
|
2 | null | 2024-04-23T15:15:35.567Z | https://wasp-lang.dev/docs/0.11.8/tutorial/pages | https://wasp-lang.dev/docs/0.11.8 | http | ## 3\. Pages & Routes
In the default `main.wasp` file created by `wasp new`, there is a **page** and a **route** declaration:
* JavaScript
* TypeScript
main.wasp
```
route RootRoute { path: "/", to: MainPage }page MainPage { // We specify that the React implementation of the page is the default export // of `src/client/MainPage.jsx`. This statement uses standard JS import syntax. // Use `@client` to reference files inside the `src/client` folder. component: import Main from "@client/MainPage.jsx"}
```
Together, these declarations tell Wasp that when a user navigates to `/`, it should render the default export from `src/client/MainPage`.
## The MainPage Component[](#the-mainpage-component "Direct link to The MainPage Component")
Let's take a look at the React component referenced by the page declaration:
* JavaScript
* TypeScript
src/client/MainPage.jsx
```
import waspLogo from './waspLogo.png'import './Main.css'const MainPage = () => { // ...}export default MainPage
```
Since Wasp uses React for the frontend, this is a normal functional React component. It also uses the CSS and logo image that are located next to it in the `src/client` folder.
That is all the code you need! Wasp takes care of everything else necessary to define, build, and run the web app.
tip
`wasp start` automatically picks up the changes you make and restarts the app, so keep it running in the background.
## Adding a Second Page[](#adding-a-second-page "Direct link to Adding a Second Page")
To add more pages, you can create another set of **page** and **route** declarations. You can even add parameters to the URL path, using the same syntax as [React Router](https://reactrouter.com/web/). Let's test this out by adding a new page:
* JavaScript
* TypeScript
main.wasp
```
route HelloRoute { path: "/hello/:name", to: HelloPage }page HelloPage { component: import Hello from "@client/HelloPage.jsx"}
```
When a user visits `/hello/their-name`, Wasp will render the component exported from `src/client/HelloPage` and pass the URL parameter the same way as in React Router:
* JavaScript
* TypeScript
src/client/HelloPage.jsx
```
const HelloPage = (props) => { return <div>Here's {props.match.params.name}!</div>}export default HelloPage
```
Now you can visit `/hello/johnny` and see "Here's johnny!"
## Cleaning Up[](#cleaning-up "Direct link to Cleaning Up")
Let's prepare for building the Todo app by cleaning up the project and removing files and code we won't need. Start by deleting `Main.css`, `waspLogo.png`, and `HelloPage.tsx` that we just created in the `src/client/` folder.
Since we deleted `HelloPage.tsx`, we also need to remember to remove the `route` and `page` declarations we wrote for it. Your Wasp file should now look like this:
* JavaScript
* TypeScript
main.wasp
```
app TodoApp { wasp: { version: "^0.11.0" }, title: "Todo app"}route RootRoute { path: "/", to: MainPage }page MainPage { component: import Main from "@client/MainPage.jsx"}
```
Next, we'll remove most of the code from the `MainPage` component:
* JavaScript
* TypeScript
src/client/MainPage.jsx
```
const MainPage = () => { return <div>Hello world!</div>}export default MainPage
```
At this point, the main page should look like this:
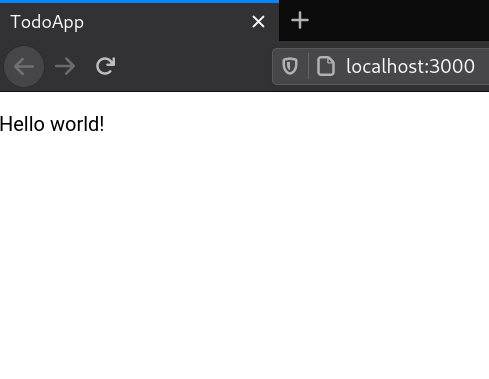
In the next section, we'll start implementing some features of the Todo app! | null | https://wasp-lang.dev/docs/0.11.8/tutorial/pages | In the default main.wasp file created by wasp new, there is a page and a route declaration: | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf526d3e5b10-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:35 GMT | Tue, 23 Apr 2024 15:25:35 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=F4bM%2BA4GXzYkiIYEsIcWTCmTDKDKtnKkUuGMxwOn%2FlAm8lraxicvpWm%2FNvE79I%2FdEgigYolDLyTQn0UCNkUFoyGc8EuhMvUbXpg%2B4FcHqeXYiNOyH%2BYQuOuYo7DwuRRx"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | c34c8db6026066b7ad2157db5c761e1f0e187c22 | null | CEBC:173D:DBA5E:10BB76:6627D097 | HIT | MISS | cache-iad-kiad7000071-IAD | S1713885335.441544,VS0,VE14 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/tutorial/pages | og:url | 3. Pages & Routes | Wasp | og:title | In the default main.wasp file created by wasp new, there is a page and a route declaration: | og:description | 3. Pages & Routes | Wasp | null | 3. Pages & Routes
In the default main.wasp file created by wasp new, there is a page and a route declaration:
JavaScript
TypeScript
main.wasp
route RootRoute { path: "/", to: MainPage }
page MainPage {
// We specify that the React implementation of the page is the default export
// of `src/client/MainPage.jsx`. This statement uses standard JS import syntax.
// Use `@client` to reference files inside the `src/client` folder.
component: import Main from "@client/MainPage.jsx"
}
Together, these declarations tell Wasp that when a user navigates to /, it should render the default export from src/client/MainPage.
The MainPage Component
Let's take a look at the React component referenced by the page declaration:
JavaScript
TypeScript
src/client/MainPage.jsx
import waspLogo from './waspLogo.png'
import './Main.css'
const MainPage = () => {
// ...
}
export default MainPage
Since Wasp uses React for the frontend, this is a normal functional React component. It also uses the CSS and logo image that are located next to it in the src/client folder.
That is all the code you need! Wasp takes care of everything else necessary to define, build, and run the web app.
tip
wasp start automatically picks up the changes you make and restarts the app, so keep it running in the background.
Adding a Second Page
To add more pages, you can create another set of page and route declarations. You can even add parameters to the URL path, using the same syntax as React Router. Let's test this out by adding a new page:
JavaScript
TypeScript
main.wasp
route HelloRoute { path: "/hello/:name", to: HelloPage }
page HelloPage {
component: import Hello from "@client/HelloPage.jsx"
}
When a user visits /hello/their-name, Wasp will render the component exported from src/client/HelloPage and pass the URL parameter the same way as in React Router:
JavaScript
TypeScript
src/client/HelloPage.jsx
const HelloPage = (props) => {
return <div>Here's {props.match.params.name}!</div>
}
export default HelloPage
Now you can visit /hello/johnny and see "Here's johnny!"
Cleaning Up
Let's prepare for building the Todo app by cleaning up the project and removing files and code we won't need. Start by deleting Main.css, waspLogo.png, and HelloPage.tsx that we just created in the src/client/ folder.
Since we deleted HelloPage.tsx, we also need to remember to remove the route and page declarations we wrote for it. Your Wasp file should now look like this:
JavaScript
TypeScript
main.wasp
app TodoApp {
wasp: {
version: "^0.11.0"
},
title: "Todo app"
}
route RootRoute { path: "/", to: MainPage }
page MainPage {
component: import Main from "@client/MainPage.jsx"
}
Next, we'll remove most of the code from the MainPage component:
JavaScript
TypeScript
src/client/MainPage.jsx
const MainPage = () => {
return <div>Hello world!</div>
}
export default MainPage
At this point, the main page should look like this:
In the next section, we'll start implementing some features of the Todo app! | https://wasp-lang.dev/docs/0.11.8/tutorial/pages |
|
2 | null | 2024-04-23T15:15:43.021Z | https://wasp-lang.dev/docs/0.11.8/tutorial/queries | https://wasp-lang.dev/docs/0.11.8 | http | ## 5\. Querying the Database
We want to know which tasks we need to do, so let's list them! The primary way of interacting with entities in Wasp is by using [queries and actions](https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview), collectively known as _operations_.
Queries are used to read an entity, while actions are used to create, modify, and delete entities. Since we want to list the tasks, we'll want to use a query.
To list tasks we have to:
1. Create a query that fetches tasks from the database.
2. Update the `MainPage.tsx` to use that query and display the results.
## Defining the Query[](#defining-the-query "Direct link to Defining the Query")
We'll create a new query called `getTasks`. We'll need to declare the query in the Wasp file and write its implementation in .
### Declaring a Query[](#declaring-a-query "Direct link to Declaring a Query")
We need to add a **query** declaration to `main.wasp` so that Wasp knows it exists:
* JavaScript
* TypeScript
main.wasp
```
// ...query getTasks { // Specifies where the implementation for the query function is. // Use `@server` to import files inside the `src/server` folder. fn: import { getTasks } from "@server/queries.js", // Tell Wasp that this query reads from the `Task` entity. By doing this, Wasp // will automatically update the results of this query when tasks are modified. entities: [Task]}
```
### Implementing a Query[](#implementing-a-query "Direct link to Implementing a Query")
* JavaScript
* TypeScript
src/server/queries.js
```
export const getTasks = async (args, context) => { return context.entities.Task.findMany({ orderBy: { id: 'asc' }, })}
```
Query function parameters:
* `args`: `object`, arguments the query is given by the caller.
* `context`: `object`, information provided by Wasp.
Since we declared in `main.wasp` that our query uses the `Task` entity, Wasp injected a [Prisma client](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-client/crud) for the `Task` entity as `context.entities.Task` - we used it above to fetch all the tasks from the database.
info
Queries and actions are NodeJS functions that are executed on the server. Therefore, we put them in the `src/server` folder.
## Invoking the Query On the Frontend[](#invoking-the-query-on-the-frontend "Direct link to Invoking the Query On the Frontend")
While we implement queries on the server, Wasp generates client-side functions that automatically takes care of serialization, network calls, and chache invalidation, allowing you to call the server code like it's a regular function. This makes it easy for us to use the `getTasks` query we just created in our React component:
* JavaScript
* TypeScript
src/client/MainPage.jsx
```
import getTasks from '@wasp/queries/getTasks'import { useQuery } from '@wasp/queries'const MainPage = () => { const { data: tasks, isLoading, error } = useQuery(getTasks) return ( <div> {tasks && <TasksList tasks={tasks} />} {isLoading && 'Loading...'} {error && 'Error: ' + error} </div> )}const Task = ({ task }) => { return ( <div> <input type="checkbox" id={String(task.id)} checked={task.isDone} /> {task.description} </div> )}const TasksList = ({ tasks }) => { if (!tasks?.length) return <div>No tasks</div> return ( <div> {tasks.map((task, idx) => ( <Task task={task} key={idx} /> ))} </div> )}export default MainPage
```
Most of this code is regular React, the only exception being the special `@wasp` imports:
We could have called the query directly using `getTasks()`, but the `useQuery` hook makes it reactive: React will re-render the component every time the query changes. Remember that Wasp automatically refreshes queries whenever the data is modified.
With these changes, you should be seeing the text "No tasks" on the screen:
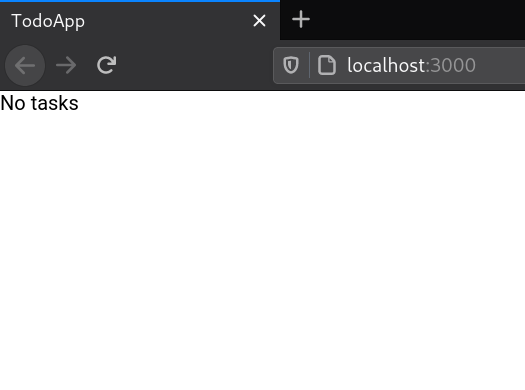
We'll create a form to add tasks in the next step 🪄 | null | https://wasp-lang.dev/docs/0.11.8/tutorial/queries | We want to know which tasks we need to do, so let's list them! The primary way of interacting with entities in Wasp is by using queries and actions, collectively known as operations. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf80a995576a-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:42 GMT | Tue, 23 Apr 2024 15:25:42 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=71qouXCotuKmdwl6%2B3CdPBanYn46baVFsMKgXPquLaacxIHkZJjqwg8XWo1Fm6O4rDZrLiZL%2BF2BujG1%2BtUFXbxo7DscoVSPotChNagX%2F%2FoWLdynv29QWHOwnYHn8LIN"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 17345038ca36fdf54940088f171e8dfc11b92303 | null | A332:16D7:4875D1:55908D:6627D09E | HIT | MISS | cache-iad-kiad7000138-IAD | S1713885343.838777,VS0,VE13 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/tutorial/queries | og:url | 5. Querying the Database | Wasp | og:title | We want to know which tasks we need to do, so let's list them! The primary way of interacting with entities in Wasp is by using queries and actions, collectively known as operations. | og:description | 5. Querying the Database | Wasp | null | 5. Querying the Database
We want to know which tasks we need to do, so let's list them! The primary way of interacting with entities in Wasp is by using queries and actions, collectively known as operations.
Queries are used to read an entity, while actions are used to create, modify, and delete entities. Since we want to list the tasks, we'll want to use a query.
To list tasks we have to:
Create a query that fetches tasks from the database.
Update the MainPage.tsx to use that query and display the results.
Defining the Query
We'll create a new query called getTasks. We'll need to declare the query in the Wasp file and write its implementation in .
Declaring a Query
We need to add a query declaration to main.wasp so that Wasp knows it exists:
JavaScript
TypeScript
main.wasp
// ...
query getTasks {
// Specifies where the implementation for the query function is.
// Use `@server` to import files inside the `src/server` folder.
fn: import { getTasks } from "@server/queries.js",
// Tell Wasp that this query reads from the `Task` entity. By doing this, Wasp
// will automatically update the results of this query when tasks are modified.
entities: [Task]
}
Implementing a Query
JavaScript
TypeScript
src/server/queries.js
export const getTasks = async (args, context) => {
return context.entities.Task.findMany({
orderBy: { id: 'asc' },
})
}
Query function parameters:
args: object, arguments the query is given by the caller.
context: object, information provided by Wasp.
Since we declared in main.wasp that our query uses the Task entity, Wasp injected a Prisma client for the Task entity as context.entities.Task - we used it above to fetch all the tasks from the database.
info
Queries and actions are NodeJS functions that are executed on the server. Therefore, we put them in the src/server folder.
Invoking the Query On the Frontend
While we implement queries on the server, Wasp generates client-side functions that automatically takes care of serialization, network calls, and chache invalidation, allowing you to call the server code like it's a regular function. This makes it easy for us to use the getTasks query we just created in our React component:
JavaScript
TypeScript
src/client/MainPage.jsx
import getTasks from '@wasp/queries/getTasks'
import { useQuery } from '@wasp/queries'
const MainPage = () => {
const { data: tasks, isLoading, error } = useQuery(getTasks)
return (
<div>
{tasks && <TasksList tasks={tasks} />}
{isLoading && 'Loading...'}
{error && 'Error: ' + error}
</div>
)
}
const Task = ({ task }) => {
return (
<div>
<input type="checkbox" id={String(task.id)} checked={task.isDone} />
{task.description}
</div>
)
}
const TasksList = ({ tasks }) => {
if (!tasks?.length) return <div>No tasks</div>
return (
<div>
{tasks.map((task, idx) => (
<Task task={task} key={idx} />
))}
</div>
)
}
export default MainPage
Most of this code is regular React, the only exception being the special @wasp imports:
We could have called the query directly using getTasks(), but the useQuery hook makes it reactive: React will re-render the component every time the query changes. Remember that Wasp automatically refreshes queries whenever the data is modified.
With these changes, you should be seeing the text "No tasks" on the screen:
We'll create a form to add tasks in the next step 🪄 | https://wasp-lang.dev/docs/0.11.8/tutorial/queries |
|
2 | null | 2024-04-23T15:15:42.923Z | https://wasp-lang.dev/docs/0.11.8/tutorial/auth | https://wasp-lang.dev/docs/0.11.8 | http | ## 7\. Adding Authentication
Most apps today require some sort of registration and login flow, so Wasp has first-class support for it. Let's add it to our Todo app!
First, we'll create a Todo list for what needs to be done (luckily we have an app for this now 😄).
* Create a `User` entity.
* Tell Wasp to use the username and password authentication.
* Add login and signup pages.
* Update the main page to require authentication.
* Add a relation between `User` and `Task` entities.
* Modify our queries and actions so that users can only see and modify their tasks.
* Add a logout button.
## Creating a User Entity[](#creating-a-user-entity "Direct link to Creating a User Entity")
Since Wasp manages authentication, it expects certain fields to exist on the `User` entity. Specifically, it expects a unique `username` field and a `password` field, both of which should be strings.
main.wasp
```
// ...entity User {=psl id Int @id @default(autoincrement()) username String @unique password Stringpsl=}
```
As we talked about earlier, we have to remember to update the database schema:
## Adding Auth to the Project[](#adding-auth-to-the-project "Direct link to Adding Auth to the Project")
Next, we want to tell Wasp that we want to use full-stack [authentication](https://wasp-lang.dev/docs/0.11.8/auth/overview) in our app:
main.wasp
```
app TodoApp { wasp: { version: "^0.11.0" }, title: "Todo app", auth: { // Tells Wasp which entity to use for storing users. userEntity: User, methods: { // Enable username and password auth. usernameAndPassword: {} }, // We'll see how this is used a bit later. onAuthFailedRedirectTo: "/login" }}// ...
```
By doing this, Wasp will create:
* [Auth UI](https://wasp-lang.dev/docs/0.11.8/auth/ui) with login and signup forms.
* A `logout()` action.
* A React hook `useAuth()`.
* `context.user` for use in Queries and Actions.
info
Wasp also supports authentication using [Google](https://wasp-lang.dev/docs/0.11.8/auth/social-auth/google), [GitHub](https://wasp-lang.dev/docs/0.11.8/auth/social-auth/github), and [email](https://wasp-lang.dev/docs/0.11.8/auth/email), with more on the way!
## Adding Login and Signup Pages[](#adding-login-and-signup-pages "Direct link to Adding Login and Signup Pages")
Wasp creates the login and signup forms for us, but we still need to define the pages to display those forms on. We'll start by declaring the pages in the Wasp file:
* JavaScript
* TypeScript
main.wasp
```
// ...route SignupRoute { path: "/signup", to: SignupPage }page SignupPage { component: import Signup from "@client/SignupPage.jsx"}route LoginRoute { path: "/login", to: LoginPage }page LoginPage { component: import Login from "@client/LoginPage.jsx"}
```
Great, Wasp now knows these pages exist! Now, the React code for the pages:
* JavaScript
* TypeScript
src/client/LoginPage.jsx
```
import { Link } from 'react-router-dom'import { LoginForm } from '@wasp/auth/forms/Login'const LoginPage = () => { return ( <div style={{ maxWidth: '400px', margin: '0 auto' }}> <LoginForm /> <br /> <span> I don't have an account yet (<Link to="/signup">go to signup</Link>). </span> </div> )}export default LoginPage
```
The Signup page is very similar to the login one:
* JavaScript
* TypeScript
src/client/SignupPage.jsx
```
import { Link } from 'react-router-dom'import { SignupForm } from '@wasp/auth/forms/Signup'const SignupPage = () => { return ( <div style={{ maxWidth: '400px', margin: '0 auto' }}> <SignupForm /> <br /> <span> I already have an account (<Link to="/login">go to login</Link>). </span> </div> )}export default SignupPage
```
## Update the Main Page to Require Auth[](#update-the-main-page-to-require-auth "Direct link to Update the Main Page to Require Auth")
We don't want users who are not logged in to access the main page, because they won't be able to create any tasks. So let's make the page private by requiring the user to be logged in:
main.wasp
```
// ...page MainPage { authRequired: true, component: import Main from "@client/MainPage"}
```
Now that auth is required for this page, unauthenticated users will be redirected to `/login`, as we specified with `app.auth.onAuthFailedRedirectTo`.
Additionally, when `authRequired` is `true`, the page's React component will be provided a `user` object as prop.
* JavaScript
* TypeScript
src/client/MainPage.jsx
```
const MainPage = ({ user }) => { // Do something with the user}
```
Ok, time to test this out. Navigate to the main page (`/`) of the app. You'll get redirected to `/login`, where you'll be asked to authenticate.
Since we just added users, you don't have an account yet. Go to the signup page and create one. You'll be sent back to the main page where you will now be able to see the TODO list!
Let's check out what the database looks like. Start the Prisma Studio:
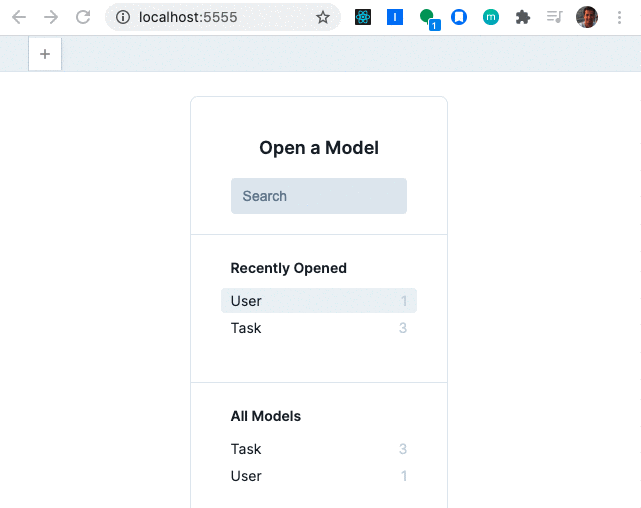
We see there is a user and that its password is already hashed 🤯
However, you will notice that if you try logging in as different users and creating some tasks, all users share the same tasks. That's because we haven't yet updated the queries and actions to have per-user tasks. Let's do that next.
## Defining a User-Task Relation[](#defining-a-user-task-relation "Direct link to Defining a User-Task Relation")
First, let's define a one-to-many relation between users and tasks (check the [Prisma docs on relations](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-schema/relations)):
main.wasp
```
// ...entity User {=psl id Int @id @default(autoincrement()) username String @unique password String tasks Task[]psl=}entity Task {=psl id Int @id @default(autoincrement()) description String isDone Boolean @default(false) user User? @relation(fields: [userId], references: [id]) userId Int?psl=}// ...
```
As always, we have to update the database:
note
We made `user` and `userId` in `Task` optional (via `?`) because that allows us to keep the existing tasks, which don't have a user assigned, in the database. This is not recommended because it allows an unwanted state in the database (what is the purpose of the task not belonging to anybody?) and normally we would not make these fields optional. Instead, we would do a data migration to take care of those tasks, even if it means just deleting them all. However, for this tutorial, for the sake of simplicity, we will stick with this.
## Updating Operations to Check Authentication[](#updating-operations-to-check-authentication "Direct link to Updating Operations to Check Authentication")
Next, let's update the queries and actions to forbid access to non-authenticated users and to operate only on the currently logged-in user's tasks:
* JavaScript
* TypeScript
src/server/queries.js
```
import HttpError from '@wasp/core/HttpError.js'export const getTasks = async (args, context) => { if (!context.user) { throw new HttpError(401) } return context.entities.Task.findMany({ where: { user: { id: context.user.id } }, orderBy: { id: 'asc' }, })}
```
* JavaScript
* TypeScript
src/server/actions.js
```
import HttpError from '@wasp/core/HttpError.js'export const createTask = async (args, context) => { if (!context.user) { throw new HttpError(401) } return context.entities.Task.create({ data: { description: args.description, user: { connect: { id: context.user.id } }, }, })}export const updateTask = async (args, context) => { if (!context.user) { throw new HttpError(401) } return context.entities.Task.updateMany({ where: { id: args.id, user: { id: context.user.id } }, data: { isDone: args.isDone }, })}
```
note
Due to how Prisma works, we had to convert `update` to `updateMany` in `updateTask` action to be able to specify the user id in `where`.
With these changes, each user should have a list of tasks that only they can see and edit.
Try playing around, adding a few users and some tasks for each of them. Then open the DB studio:
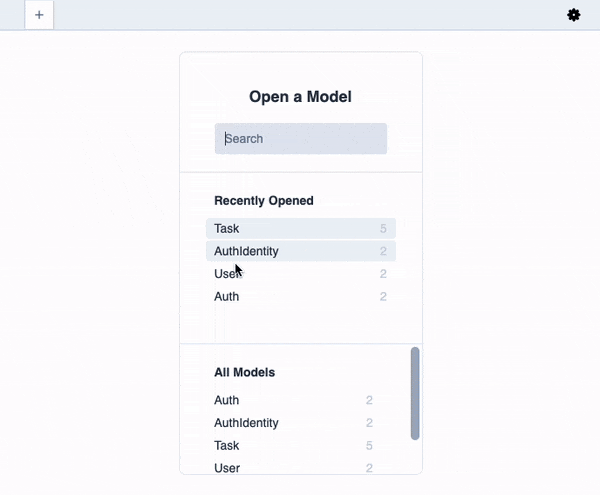
You will see that each user has their tasks, just as we specified in our code!
## Logout Button[](#logout-button "Direct link to Logout Button")
Last, but not least, let's add the logout functionality:
* JavaScript
* TypeScript
src/client/MainPage.jsx
```
// ...import logout from '@wasp/auth/logout'//...const MainPage = () => { // ... return ( <div> // ... <button onClick={logout}>Logout</button> </div> )}
```
This is it, we have a working authentication system, and our Todo app is multi-user!
## What's Next?[](#whats-next "Direct link to What's Next?")
We did it 🎉 You've followed along with this tutorial to create a basic Todo app with Wasp.
You should be ready to learn about more complicated features and go more in-depth with the features already covered. Scroll through the sidebar on the left side of the page to see every feature Wasp has to offer. Or, let your imagination run wild and start building your app! ✨
Looking for inspiration?
* Get a jump start on your next project with [Starter Templates](https://wasp-lang.dev/docs/0.11.8/project/starter-templates)
* Make a real-time app with [Web Sockets](https://wasp-lang.dev/docs/0.11.8/advanced/web-sockets)
note
If you notice that some of the features you'd like to have are missing, or have any other kind of feedback, please write to us on [Discord](https://discord.gg/rzdnErX) or create an issue on [Github](https://github.com/wasp-lang/wasp), so we can learn which features to add/improve next 🙏
If you would like to contribute or help to build a feature, let us know! You can find more details on contributing [here](https://wasp-lang.dev/docs/0.11.8/contributing).
Oh, and do [**subscribe to our newsletter**](https://wasp-lang.dev/#signup)! We usually send one per month, and Matija does his best to unleash his creativity to make them engaging and fun to read :D! | null | https://wasp-lang.dev/docs/0.11.8/tutorial/auth | Most apps today require some sort of registration and login flow, so Wasp has first-class support for it. Let's add it to our Todo app! | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf801cca72e7-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:42 GMT | Tue, 23 Apr 2024 15:25:42 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=IouJURgMOBD%2Bw8YgUbNmlgCZfxL1Srfv82z2jr1ZuAwVrt7vKfUO52qBEmao2gyPBWy5w2ve0rl9G4F8H2XFoyyg%2FDTL6NoickNi3EBbsnyTxE332snaU8SwttnI8t8r"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | f3e4a0af0510b864e5f25888171b46b1bdec2082 | null | 75A6:277B7:3B378E5:4654A77:6627D09E | HIT | MISS | cache-iad-kiad7000179-IAD | S1713885343.753436,VS0,VE18 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/tutorial/auth | og:url | 7. Adding Authentication | Wasp | og:title | Most apps today require some sort of registration and login flow, so Wasp has first-class support for it. Let's add it to our Todo app! | og:description | 7. Adding Authentication | Wasp | null | 7. Adding Authentication
Most apps today require some sort of registration and login flow, so Wasp has first-class support for it. Let's add it to our Todo app!
First, we'll create a Todo list for what needs to be done (luckily we have an app for this now 😄).
Create a User entity.
Tell Wasp to use the username and password authentication.
Add login and signup pages.
Update the main page to require authentication.
Add a relation between User and Task entities.
Modify our queries and actions so that users can only see and modify their tasks.
Add a logout button.
Creating a User Entity
Since Wasp manages authentication, it expects certain fields to exist on the User entity. Specifically, it expects a unique username field and a password field, both of which should be strings.
main.wasp
// ...
entity User {=psl
id Int @id @default(autoincrement())
username String @unique
password String
psl=}
As we talked about earlier, we have to remember to update the database schema:
Adding Auth to the Project
Next, we want to tell Wasp that we want to use full-stack authentication in our app:
main.wasp
app TodoApp {
wasp: {
version: "^0.11.0"
},
title: "Todo app",
auth: {
// Tells Wasp which entity to use for storing users.
userEntity: User,
methods: {
// Enable username and password auth.
usernameAndPassword: {}
},
// We'll see how this is used a bit later.
onAuthFailedRedirectTo: "/login"
}
}
// ...
By doing this, Wasp will create:
Auth UI with login and signup forms.
A logout() action.
A React hook useAuth().
context.user for use in Queries and Actions.
info
Wasp also supports authentication using Google, GitHub, and email, with more on the way!
Adding Login and Signup Pages
Wasp creates the login and signup forms for us, but we still need to define the pages to display those forms on. We'll start by declaring the pages in the Wasp file:
JavaScript
TypeScript
main.wasp
// ...
route SignupRoute { path: "/signup", to: SignupPage }
page SignupPage {
component: import Signup from "@client/SignupPage.jsx"
}
route LoginRoute { path: "/login", to: LoginPage }
page LoginPage {
component: import Login from "@client/LoginPage.jsx"
}
Great, Wasp now knows these pages exist! Now, the React code for the pages:
JavaScript
TypeScript
src/client/LoginPage.jsx
import { Link } from 'react-router-dom'
import { LoginForm } from '@wasp/auth/forms/Login'
const LoginPage = () => {
return (
<div style={{ maxWidth: '400px', margin: '0 auto' }}>
<LoginForm />
<br />
<span>
I don't have an account yet (<Link to="/signup">go to signup</Link>).
</span>
</div>
)
}
export default LoginPage
The Signup page is very similar to the login one:
JavaScript
TypeScript
src/client/SignupPage.jsx
import { Link } from 'react-router-dom'
import { SignupForm } from '@wasp/auth/forms/Signup'
const SignupPage = () => {
return (
<div style={{ maxWidth: '400px', margin: '0 auto' }}>
<SignupForm />
<br />
<span>
I already have an account (<Link to="/login">go to login</Link>).
</span>
</div>
)
}
export default SignupPage
Update the Main Page to Require Auth
We don't want users who are not logged in to access the main page, because they won't be able to create any tasks. So let's make the page private by requiring the user to be logged in:
main.wasp
// ...
page MainPage {
authRequired: true,
component: import Main from "@client/MainPage"
}
Now that auth is required for this page, unauthenticated users will be redirected to /login, as we specified with app.auth.onAuthFailedRedirectTo.
Additionally, when authRequired is true, the page's React component will be provided a user object as prop.
JavaScript
TypeScript
src/client/MainPage.jsx
const MainPage = ({ user }) => {
// Do something with the user
}
Ok, time to test this out. Navigate to the main page (/) of the app. You'll get redirected to /login, where you'll be asked to authenticate.
Since we just added users, you don't have an account yet. Go to the signup page and create one. You'll be sent back to the main page where you will now be able to see the TODO list!
Let's check out what the database looks like. Start the Prisma Studio:
We see there is a user and that its password is already hashed 🤯
However, you will notice that if you try logging in as different users and creating some tasks, all users share the same tasks. That's because we haven't yet updated the queries and actions to have per-user tasks. Let's do that next.
Defining a User-Task Relation
First, let's define a one-to-many relation between users and tasks (check the Prisma docs on relations):
main.wasp
// ...
entity User {=psl
id Int @id @default(autoincrement())
username String @unique
password String
tasks Task[]
psl=}
entity Task {=psl
id Int @id @default(autoincrement())
description String
isDone Boolean @default(false)
user User? @relation(fields: [userId], references: [id])
userId Int?
psl=}
// ...
As always, we have to update the database:
note
We made user and userId in Task optional (via ?) because that allows us to keep the existing tasks, which don't have a user assigned, in the database. This is not recommended because it allows an unwanted state in the database (what is the purpose of the task not belonging to anybody?) and normally we would not make these fields optional. Instead, we would do a data migration to take care of those tasks, even if it means just deleting them all. However, for this tutorial, for the sake of simplicity, we will stick with this.
Updating Operations to Check Authentication
Next, let's update the queries and actions to forbid access to non-authenticated users and to operate only on the currently logged-in user's tasks:
JavaScript
TypeScript
src/server/queries.js
import HttpError from '@wasp/core/HttpError.js'
export const getTasks = async (args, context) => {
if (!context.user) {
throw new HttpError(401)
}
return context.entities.Task.findMany({
where: { user: { id: context.user.id } },
orderBy: { id: 'asc' },
})
}
JavaScript
TypeScript
src/server/actions.js
import HttpError from '@wasp/core/HttpError.js'
export const createTask = async (args, context) => {
if (!context.user) {
throw new HttpError(401)
}
return context.entities.Task.create({
data: {
description: args.description,
user: { connect: { id: context.user.id } },
},
})
}
export const updateTask = async (args, context) => {
if (!context.user) {
throw new HttpError(401)
}
return context.entities.Task.updateMany({
where: { id: args.id, user: { id: context.user.id } },
data: { isDone: args.isDone },
})
}
note
Due to how Prisma works, we had to convert update to updateMany in updateTask action to be able to specify the user id in where.
With these changes, each user should have a list of tasks that only they can see and edit.
Try playing around, adding a few users and some tasks for each of them. Then open the DB studio:
You will see that each user has their tasks, just as we specified in our code!
Logout Button
Last, but not least, let's add the logout functionality:
JavaScript
TypeScript
src/client/MainPage.jsx
// ...
import logout from '@wasp/auth/logout'
//...
const MainPage = () => {
// ...
return (
<div>
// ...
<button onClick={logout}>Logout</button>
</div>
)
}
This is it, we have a working authentication system, and our Todo app is multi-user!
What's Next?
We did it 🎉 You've followed along with this tutorial to create a basic Todo app with Wasp.
You should be ready to learn about more complicated features and go more in-depth with the features already covered. Scroll through the sidebar on the left side of the page to see every feature Wasp has to offer. Or, let your imagination run wild and start building your app! ✨
Looking for inspiration?
Get a jump start on your next project with Starter Templates
Make a real-time app with Web Sockets
note
If you notice that some of the features you'd like to have are missing, or have any other kind of feedback, please write to us on Discord or create an issue on Github, so we can learn which features to add/improve next 🙏
If you would like to contribute or help to build a feature, let us know! You can find more details on contributing here.
Oh, and do subscribe to our newsletter! We usually send one per month, and Matija does his best to unleash his creativity to make them engaging and fun to read :D! | https://wasp-lang.dev/docs/0.11.8/tutorial/auth |
|
2 | 200 | 2024-04-23T15:15:39.015Z | https://wasp-lang.dev/docs/0.11.8/tutorial/create | https://wasp-lang.dev/docs/0.11.8 | browser | info
You'll need to have the latest version of Wasp installed locally to follow this tutorial. If you haven't installed it yet, check out the [QuickStart](https://wasp-lang.dev/docs/0.11.8/quick-start) guide!
In this section, we'll guide you through the process of creating a simple Todo app with Wasp. In the process, we'll take you through the most important and useful features of Wasp.
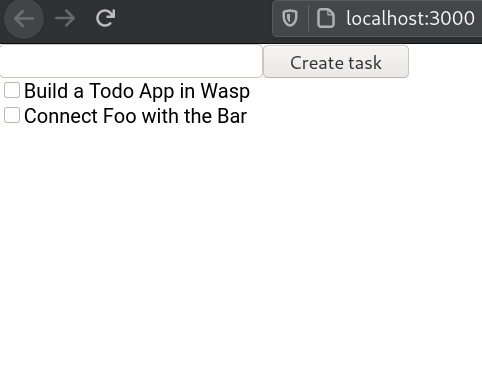
If you get stuck at any point (or just want to chat), reach out to us on [Discord](https://discord.gg/rzdnErX) and we will help you!
You can find the complete code of the app we're about to build [here](https://github.com/wasp-lang/wasp/tree/release/examples/tutorials/TodoApp).
See Wasp In Action
Prefer videos? We have a YouTube tutorial whick walks you through building this Todo app step by step. [Check it out here!](https://youtu.be/R8uOu6ZEr5s).
We've also set up an in-browser dev environment for you on Gitpod which allows you to view and edit the completed app with no installation required.
[](https://gitpod.io/#https://github.com/wasp-lang/gitpod-template)
## Creating a Project[](#creating-a-project "Direct link to Creating a Project")
To setup a new Wasp project, run the following command in your terminal
Enter the newly created directory and start the development server:
note
`wasp start` will take a bit of time to start the server the first time you run it in a new project.
You will see log messages from the client, server, and database setting themselves up. When everything is ready, a new tab should open in your browser at `http://localhost:3000` with a simple placeholder plage:
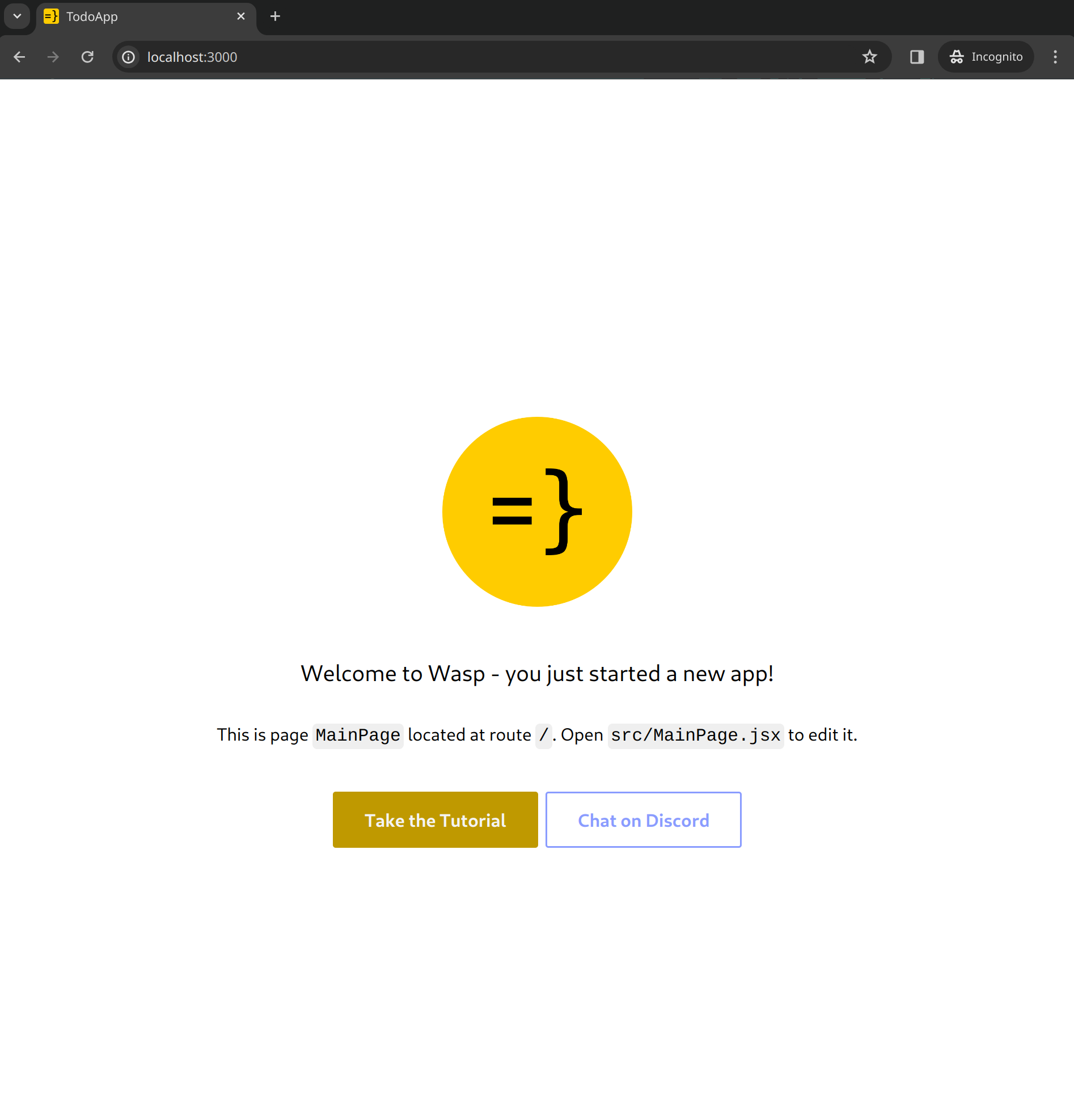
Wasp has generated for you the full front-end and back-end code the app! Next, we'll take a closer look at how the project is structured. | null | https://wasp-lang.dev/docs/0.11.8/tutorial/create | You'll need to have the latest version of Wasp installed locally to follow this tutorial. If you haven't installed it yet, check out the QuickStart guide! | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf521ac04bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:35 GMT | Tue, 23 Apr 2024 15:25:35 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=TJVOPe6Jv15t1Fg4I46B7NJQaBQmoGyCNOaRw50NLKj%2FwYteP%2FUPdXQ6UlHputGFfpv6g4bjnw12jF5iC9hhEZ2%2B9DuUWozjbIFMsm0BS7Aw4QSeGboH%2BVuyBS4H1eex"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | db745fcd2ed7f03461cdf87be3a5b41ff8dd571e | h2 | D768:16C4F4:3910116:44163E3:6627D097 | HIT | MISS | cache-nyc-kteb1890087-NYC | S1713885335.385723, VS0, VE19 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/tutorial/create | og:url | 1. Creating a New Project | Wasp | og:title | You'll need to have the latest version of Wasp installed locally to follow this tutorial. If you haven't installed it yet, check out the QuickStart guide! | og:description | 1. Creating a New Project | Wasp | null | info
You'll need to have the latest version of Wasp installed locally to follow this tutorial. If you haven't installed it yet, check out the QuickStart guide!
In this section, we'll guide you through the process of creating a simple Todo app with Wasp. In the process, we'll take you through the most important and useful features of Wasp.
If you get stuck at any point (or just want to chat), reach out to us on Discord and we will help you!
You can find the complete code of the app we're about to build here.
See Wasp In Action
Prefer videos? We have a YouTube tutorial whick walks you through building this Todo app step by step. Check it out here!.
We've also set up an in-browser dev environment for you on Gitpod which allows you to view and edit the completed app with no installation required.
Creating a Project
To setup a new Wasp project, run the following command in your terminal
Enter the newly created directory and start the development server:
note
wasp start will take a bit of time to start the server the first time you run it in a new project.
You will see log messages from the client, server, and database setting themselves up. When everything is ready, a new tab should open in your browser at http://localhost:3000 with a simple placeholder plage:
Wasp has generated for you the full front-end and back-end code the app! Next, we'll take a closer look at how the project is structured. | https://wasp-lang.dev/docs/0.11.8/tutorial/create |
|
2 | null | 2024-04-23T15:15:43.823Z | https://wasp-lang.dev/docs/0.11.8/data-model/entities | https://wasp-lang.dev/docs/0.11.8 | http | ## Entities
Entities are the foundation of your app's data model. In short, an Entity defines a model in your database.
Wasp uses the excellent [Prisma ORM](https://www.prisma.io/) to implement all database functionality and occasionally enhances it with a thin abstraction layer. Wasp Entities directly correspond to [Prisma's data model](https://www.prisma.io/docs/concepts/components/prisma-schema/data-model). Still, you don't need to be familiar with Prisma to effectively use Wasp, as it comes with a simple API wrapper for working with Prisma's core features.
The only requirement for defining Wasp Entities is familiarity with the **_Prisma Schema Language (PSL)_**, a simple definition language explicitly created for defining models in Prisma. The language is declarative and very intuitive. We'll also go through an example later in the text, so there's no need to go and thoroughly learn it right away. Still, if you're curious, look no further than Prisma's official documentation:
* [Basic intro and examples](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-schema)
* [A more exhaustive language specification](https://www.prisma.io/docs/reference/api-reference/prisma-schema-reference)
## Defining an Entity[](#defining-an-entity "Direct link to Defining an Entity")
As mentioned, an `entity` declaration represents a database model.
Each `Entity` declaration corresponds 1-to-1 to [Prisma's data model](https://www.prisma.io/docs/concepts/components/prisma-schema/data-model). Here's how you could define an Entity that represents a Task:
* JavaScript
* TypeScript
```
entity Task {=psl id Int @id @default(autoincrement()) description String isDone Boolean @default(false)psl=}
```
Let's go through this declaration in detail:
* `entity Task` - This tells Wasp that we wish to define an Entity (i.e., database model) called `Task`. Wasp automatically creates a table called `tasks`.
* `{=psl ... psl=}` - Wasp treats everything that comes between the two `psl` tags as [PSL (Prisma Schema Language)](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-schema).
The above PSL definition tells Wasp to create a table for storing Tasks where each task has three fields (i.e., the `tasks` table has three columns):
* `id` - An integer value serving as a primary key. The database automatically generates it by incrementing the previously generated `id`.
* `description` - A string value for storing the task's description.
* `isDone` - A boolean value indicating the task's completion status. If you don't set it when creating a new task, the database sets it to `false` by default.
### Working with Entities[](#working-with-entities "Direct link to Working with Entities")
Let's see how you can define and work with Wasp Entities:
1. Create/update some Entities in your `.wasp` file.
2. Run `wasp db migrate-dev`. This command syncs the database model with the Entity definitions in your `.wasp` file. It does this by creating migration scripts.
3. Migration scripts are automatically placed in the `migrations/` folder. Make sure to commit this folder into version control.
4. Use Wasp's JavasScript API to work with the database when implementing Operations (we'll cover this in detail when we talk about [operations](https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview)).
#### Using Entities in Operations[](#using-entities-in-operations "Direct link to Using Entities in Operations")
Most of the time, you will be working with Entities within the context of [Operations (Queries & Actions)](https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview). We'll see how that's done on the next page.
#### Using Entities directly[](#using-entities-directly "Direct link to Using Entities directly")
If you need more control, you can directly interact with Entities by importing and using the [Prisma Client](https://www.prisma.io/docs/concepts/components/prisma-client/crud). We recommend sticking with conventional Wasp-provided mechanisms, only resorting to directly using the Prisma client only if you need a feature Wasp doesn't provide.
You can only use the Prisma Client in your Wasp server code. You can import it like this:
* JavaScript
* TypeScript
```
import prismaClient from '@wasp/dbClient'`prismaClient.task.create({ description: "Read the Entities doc", isDone: true // almost :)})
```
### Next steps[](#next-steps "Direct link to Next steps")
Now that we've seen how to define Entities that represent Wasp's core data model, we'll see how to make the most of them in other parts of Wasp. Keep reading to learn all about Wasp Operations! | null | https://wasp-lang.dev/docs/0.11.8/data-model/entities | Entities are the foundation of your app's data model. In short, an Entity defines a model in your database. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf863f0007bc-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:43 GMT | Tue, 23 Apr 2024 15:25:43 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=oikLERVggna0hAa6yqkQ72UGUHIQqElsNMVwMWxySiXhmMsvgcw7geHSMtR0uSpX9IvHQR6iWdMh9kvQfiKskocX0n8J9qG8loEFC%2Bjou6U4zhnX%2FyQiul46rZhNWDuU"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | ed370312f1b027168972242a4c53d623053a5fa4 | null | 8C46:1736:5926FE:6B028E:6627D09F | HIT | MISS | cache-iad-kiad7000098-IAD | S1713885344.752832,VS0,VE13 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/data-model/entities | og:url | Entities | Wasp | og:title | Entities are the foundation of your app's data model. In short, an Entity defines a model in your database. | og:description | Entities | Wasp | null | Entities
Entities are the foundation of your app's data model. In short, an Entity defines a model in your database.
Wasp uses the excellent Prisma ORM to implement all database functionality and occasionally enhances it with a thin abstraction layer. Wasp Entities directly correspond to Prisma's data model. Still, you don't need to be familiar with Prisma to effectively use Wasp, as it comes with a simple API wrapper for working with Prisma's core features.
The only requirement for defining Wasp Entities is familiarity with the Prisma Schema Language (PSL), a simple definition language explicitly created for defining models in Prisma. The language is declarative and very intuitive. We'll also go through an example later in the text, so there's no need to go and thoroughly learn it right away. Still, if you're curious, look no further than Prisma's official documentation:
Basic intro and examples
A more exhaustive language specification
Defining an Entity
As mentioned, an entity declaration represents a database model.
Each Entity declaration corresponds 1-to-1 to Prisma's data model. Here's how you could define an Entity that represents a Task:
JavaScript
TypeScript
entity Task {=psl
id Int @id @default(autoincrement())
description String
isDone Boolean @default(false)
psl=}
Let's go through this declaration in detail:
entity Task - This tells Wasp that we wish to define an Entity (i.e., database model) called Task. Wasp automatically creates a table called tasks.
{=psl ... psl=} - Wasp treats everything that comes between the two psl tags as PSL (Prisma Schema Language).
The above PSL definition tells Wasp to create a table for storing Tasks where each task has three fields (i.e., the tasks table has three columns):
id - An integer value serving as a primary key. The database automatically generates it by incrementing the previously generated id.
description - A string value for storing the task's description.
isDone - A boolean value indicating the task's completion status. If you don't set it when creating a new task, the database sets it to false by default.
Working with Entities
Let's see how you can define and work with Wasp Entities:
Create/update some Entities in your .wasp file.
Run wasp db migrate-dev. This command syncs the database model with the Entity definitions in your .wasp file. It does this by creating migration scripts.
Migration scripts are automatically placed in the migrations/ folder. Make sure to commit this folder into version control.
Use Wasp's JavasScript API to work with the database when implementing Operations (we'll cover this in detail when we talk about operations).
Using Entities in Operations
Most of the time, you will be working with Entities within the context of Operations (Queries & Actions). We'll see how that's done on the next page.
Using Entities directly
If you need more control, you can directly interact with Entities by importing and using the Prisma Client. We recommend sticking with conventional Wasp-provided mechanisms, only resorting to directly using the Prisma client only if you need a feature Wasp doesn't provide.
You can only use the Prisma Client in your Wasp server code. You can import it like this:
JavaScript
TypeScript
import prismaClient from '@wasp/dbClient'`
prismaClient.task.create({
description: "Read the Entities doc",
isDone: true // almost :)
})
Next steps
Now that we've seen how to define Entities that represent Wasp's core data model, we'll see how to make the most of them in other parts of Wasp. Keep reading to learn all about Wasp Operations! | https://wasp-lang.dev/docs/0.11.8/data-model/entities |
|
2 | 200 | 2024-04-23T15:15:48.847Z | https://wasp-lang.dev/docs/0.11.8/tutorial/actions | https://wasp-lang.dev/docs/0.11.8 | browser | ## 6\. Modifying Data
In the previous section, we learned about using queries to fetch data and only briefly mentioned that actions can be used to update the database. Let's learn more about actions so we can add and update tasks in the database.
We have to create:
1. A Wasp action that creates a new task.
2. A React form that calls that action when the user creates a task.
## Creating a New Action[](#creating-a-new-action "Direct link to Creating a New Action")
Creating an action is very similar to creating a query.
### Declaring an Action[](#declaring-an-action "Direct link to Declaring an Action")
We must first declare the action in `main.wasp`:
* JavaScript
* TypeScript
main.wasp
```
// ...action createTask { fn: import { createTask } from "@server/actions.js", entities: [Task]}
```
### Implementing an Action[](#implementing-an-action "Direct link to Implementing an Action")
Let's now define a function for our `createTask` action:
* JavaScript
* TypeScript
src/server/actions.js
```
export const createTask = async (args, context) => { return context.entities.Task.create({ data: { description: args.description }, })}
```
tip
We put the function in a new file `src/server/actions.ts`, but we could have put it anywhere we wanted! There are no limitations here, as long as the declaration in the Wasp file imports it correctly and the file is located within `src/server`.
## Invoking the Action on the Client[](#invoking-the-action-on-the-client "Direct link to Invoking the Action on the Client")
First, let's define a form that the user can create new tasks with.
* JavaScript
* TypeScript
src/client/MainPage.jsx
```
import getTasks from '@wasp/queries/getTasks'import createTask from '@wasp/actions/createTask'import { useQuery } from '@wasp/queries'// ...const NewTaskForm = () => { const handleSubmit = async (event) => { event.preventDefault() try { const target = event.target const description = target.description.value target.reset() await createTask({ description }) } catch (err) { window.alert('Error: ' + err.message) } } return ( <form onSubmit={handleSubmit}> <input name="description" type="text" defaultValue="" /> <input type="submit" value="Create task" /> </form> )}
```
Unlike queries, you call actions directly (i.e., without wrapping it with a hook) because we don't need reactivity. The rest is just regular React code.
Now, we just need to add this form to the page component:
* JavaScript
* TypeScript
src/client/MainPage.tsx
```
import getTasks from '@wasp/queries/getTasks'import createTask from '@wasp/actions/createTask'import { useQuery } from '@wasp/queries'const MainPage = () => { const { data: tasks, isLoading, error } = useQuery(getTasks) return ( <div> <NewTaskForm /> {tasks && <TasksList tasks={tasks} />} {isLoading && 'Loading...'} {error && 'Error: ' + error} </div> )}
```
And now we have a form that creates new tasks.
Try creating a "Build a Todo App in Wasp" task and see it appear in the list below. The task is created on the server and saved in the database.
Try refreshing the page or opening it in another browser, you'll see the tasks are still there!
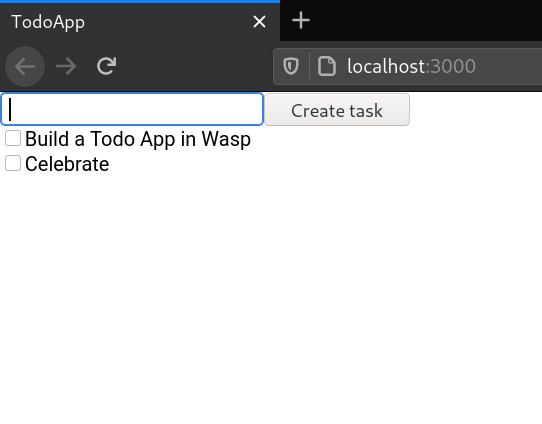
Automatic Query Invalidation
When you create a new task, the list of tasks is automatically updated to display the new task, even though we have not written any code that would do that! These automatic updates are handled by code that Wasp generates.
When you declared the `getTasks` and `createTask` operations, you specified that they both use the `Task` entity. So when `createTask` is called, Wasp knows that the data `getTasks` fetches may have changed and automatically updates it in the background. This means that **out of the box, Wasp will make sure that all your queries are kept in-sync with changes made by any actions**.
This behavior is convenient as a default but can cause poor performance in large apps. While there is no mechanism for overriding this behavior yet, it is something that we plan to include in Wasp in the future. This feature is tracked [here](https://github.com/wasp-lang/wasp/issues/63).
## A Second Action[](#a-second-action "Direct link to A Second Action")
Our Todo app isn't finished if you can't mark a task as done! We'll create a new action to update a task's status and call it from React whenever a task's checkbox is toggled.
Since we've already created one task together, try to create this one yourself. It should be an action named `updateTask` that takes a task `id` and an `isDone` in its arguments. You can check our implementation below.
Solution
Now, we can call `updateTask` from the React component:
* JavaScript
* TypeScript
src/client/MainPage.jsx
```
// ...import updateTask from '@wasp/actions/updateTask'// ...const Task = ({ task }) => { const handleIsDoneChange = async (event) => { try { await updateTask({ id: task.id, isDone: event.target.checked, }) } catch (error) { window.alert('Error while updating task: ' + error.message) } } return ( <div> <input type="checkbox" id={String(task.id)} checked={task.isDone} onChange={handleIsDoneChange} /> {task.description} </div> )}// ...
```
Awesome! Now we can check off this task 🙃 Let's add one more interesting feature to our app. | null | https://wasp-lang.dev/docs/0.11.8/tutorial/actions | In the previous section, we learned about using queries to fetch data and only briefly mentioned that actions can be used to update the database. Let's learn more about actions so we can add and update tasks in the database. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecf86fc694bcc-BUF | null | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:43 GMT | Tue, 23 Apr 2024 15:25:43 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0, "report_to":"cf-nel", "max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=SW6mtM4pW4oQeYnxc3YxEOnIo1WOdkTot3BSj5%2BE5PT%2B56hGwv3FnMKMpq7MThPtvz8oHK3YF7EwA421ExbDk1rVvXjL3yjE8%2FSC2b56vvzhZ5NjW7KWJNK4F3ub9TnC"}], "group":"cf-nel", "max_age":604800} | cloudflare | null | Accept-Encoding | 1.1 varnish | MISS | 0 | ef67e3aee0cf7d8ad019bfa9b52d80ed5acca554 | h2 | 896C:209F3A:3A8278F:45894F6:6627D09F | HIT | MISS | cache-nyc-kteb1890087-NYC | S1713885344.850023, VS0, VE21 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/tutorial/actions | og:url | 6. Modifying Data | Wasp | og:title | In the previous section, we learned about using queries to fetch data and only briefly mentioned that actions can be used to update the database. Let's learn more about actions so we can add and update tasks in the database. | og:description | 6. Modifying Data | Wasp | null | 6. Modifying Data
In the previous section, we learned about using queries to fetch data and only briefly mentioned that actions can be used to update the database. Let's learn more about actions so we can add and update tasks in the database.
We have to create:
A Wasp action that creates a new task.
A React form that calls that action when the user creates a task.
Creating a New Action
Creating an action is very similar to creating a query.
Declaring an Action
We must first declare the action in main.wasp:
JavaScript
TypeScript
main.wasp
// ...
action createTask {
fn: import { createTask } from "@server/actions.js",
entities: [Task]
}
Implementing an Action
Let's now define a function for our createTask action:
JavaScript
TypeScript
src/server/actions.js
export const createTask = async (args, context) => {
return context.entities.Task.create({
data: { description: args.description },
})
}
tip
We put the function in a new file src/server/actions.ts, but we could have put it anywhere we wanted! There are no limitations here, as long as the declaration in the Wasp file imports it correctly and the file is located within src/server.
Invoking the Action on the Client
First, let's define a form that the user can create new tasks with.
JavaScript
TypeScript
src/client/MainPage.jsx
import getTasks from '@wasp/queries/getTasks'
import createTask from '@wasp/actions/createTask'
import { useQuery } from '@wasp/queries'
// ...
const NewTaskForm = () => {
const handleSubmit = async (event) => {
event.preventDefault()
try {
const target = event.target
const description = target.description.value
target.reset()
await createTask({ description })
} catch (err) {
window.alert('Error: ' + err.message)
}
}
return (
<form onSubmit={handleSubmit}>
<input name="description" type="text" defaultValue="" />
<input type="submit" value="Create task" />
</form>
)
}
Unlike queries, you call actions directly (i.e., without wrapping it with a hook) because we don't need reactivity. The rest is just regular React code.
Now, we just need to add this form to the page component:
JavaScript
TypeScript
src/client/MainPage.tsx
import getTasks from '@wasp/queries/getTasks'
import createTask from '@wasp/actions/createTask'
import { useQuery } from '@wasp/queries'
const MainPage = () => {
const { data: tasks, isLoading, error } = useQuery(getTasks)
return (
<div>
<NewTaskForm />
{tasks && <TasksList tasks={tasks} />}
{isLoading && 'Loading...'}
{error && 'Error: ' + error}
</div>
)
}
And now we have a form that creates new tasks.
Try creating a "Build a Todo App in Wasp" task and see it appear in the list below. The task is created on the server and saved in the database.
Try refreshing the page or opening it in another browser, you'll see the tasks are still there!
Automatic Query Invalidation
When you create a new task, the list of tasks is automatically updated to display the new task, even though we have not written any code that would do that! These automatic updates are handled by code that Wasp generates.
When you declared the getTasks and createTask operations, you specified that they both use the Task entity. So when createTask is called, Wasp knows that the data getTasks fetches may have changed and automatically updates it in the background. This means that out of the box, Wasp will make sure that all your queries are kept in-sync with changes made by any actions.
This behavior is convenient as a default but can cause poor performance in large apps. While there is no mechanism for overriding this behavior yet, it is something that we plan to include in Wasp in the future. This feature is tracked here.
A Second Action
Our Todo app isn't finished if you can't mark a task as done! We'll create a new action to update a task's status and call it from React whenever a task's checkbox is toggled.
Since we've already created one task together, try to create this one yourself. It should be an action named updateTask that takes a task id and an isDone in its arguments. You can check our implementation below.
Solution
Now, we can call updateTask from the React component:
JavaScript
TypeScript
src/client/MainPage.jsx
// ...
import updateTask from '@wasp/actions/updateTask'
// ...
const Task = ({ task }) => {
const handleIsDoneChange = async (event) => {
try {
await updateTask({
id: task.id,
isDone: event.target.checked,
})
} catch (error) {
window.alert('Error while updating task: ' + error.message)
}
}
return (
<div>
<input
type="checkbox"
id={String(task.id)}
checked={task.isDone}
onChange={handleIsDoneChange}
/>
{task.description}
</div>
)
}
// ...
Awesome! Now we can check off this task 🙃 Let's add one more interesting feature to our app. | https://wasp-lang.dev/docs/0.11.8/tutorial/actions |
|
2 | null | 2024-04-23T15:15:53.128Z | https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview | https://wasp-lang.dev/docs/0.11.8 | http | Version: 0.11.8
## Overview
While Entities enable help you define your app's data model and relationships, Operations are all about working with this data.
There are two kinds of Operations: [Queries](https://wasp-lang.dev/docs/0.11.8/data-model/operations/queries) and [Actions](https://wasp-lang.dev/docs/0.11.8/data-model/operations/actions). As their names suggest, Queries are meant for reading data, and Actions are meant for changing it (either by updating existing entries or creating new ones).
Keep reading to find out all there is to know about Operations in Wasp. | null | https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview | While Entities enable help you define your app's data model and relationships, Operations are all about working with this data. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecfbfad5d8236-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:52 GMT | Tue, 23 Apr 2024 15:25:52 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=dJ4nOTTlXx4bnUPwuiC0m96oSH57owJmsqZsD8ilN0Tl1cgrOuayTbQ6gKUe6Q7K8D6PpkwqRhUy03yc8eM4syIKIvnkPW30SNahkqVB19ULf61hRQb709TQEzyH0irV"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 7d69fdb26f37c1307716d1186433b2c0582bfa1f | null | 3D42:180046:3C315E8:4738057:6627D0A8 | HIT | MISS | cache-iad-kiad7000025-IAD | S1713885353.942513,VS0,VE13 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview | og:url | Overview | Wasp | og:title | While Entities enable help you define your app's data model and relationships, Operations are all about working with this data. | og:description | Overview | Wasp | null | Version: 0.11.8
Overview
While Entities enable help you define your app's data model and relationships, Operations are all about working with this data.
There are two kinds of Operations: Queries and Actions. As their names suggest, Queries are meant for reading data, and Actions are meant for changing it (either by updating existing entries or creating new ones).
Keep reading to find out all there is to know about Operations in Wasp. | https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview |
|
2 | null | 2024-04-23T15:15:52.951Z | https://wasp-lang.dev/docs/0.11.8/data-model/crud | https://wasp-lang.dev/docs/0.11.8 | http | ## Automatic CRUD
If you have a lot of experience writing full-stack apps, you probably ended up doing some of the same things many times: listing data, adding data, editing it, and deleting it.
Wasp makes handling these boring bits easy by offering a higher-level concept called Automatic CRUD.
With a single declaration, you can tell Wasp to automatically generate server-side logic (i.e., Queries and Actions) for creating, reading, updating and deleting [Entities](https://wasp-lang.dev/docs/0.11.8/data-model/entities). As you update definitions for your Entities, Wasp automatically regenerates the backend logic.
Early preview
This feature is currently in early preview and we are actively working on it. Read more about [our plans](#future-of-crud-operations-in-wasp) for CRUD operations.
## Overview[](#overview "Direct link to Overview")
Imagine we have a `Task` entity and we want to enable CRUD operations for it.
main.wasp
```
entity Task {=psl id Int @id @default(autoincrement()) description String isDone Booleanpsl=}
```
We can then define a new `crud` called `Tasks`.
We specify to use the `Task` entity and we enable the `getAll`, `get`, `create` and `update` operations (let's say we don't need the `delete` operation).
main.wasp
```
crud Tasks { entity: Task, operations: { getAll: { isPublic: true, // by default only logged in users can perform operations }, get: {}, create: { overrideFn: import { createTask } from "@server/tasks.js", }, update: {}, },}
```
1. It uses default implementation for `getAll`, `get`, and `update`,
2. ... while specifying a custom implementation for `create`.
3. `getAll` will be public (no auth needed), while the rest of the operations will be private.
Here's what it looks like when visualized:
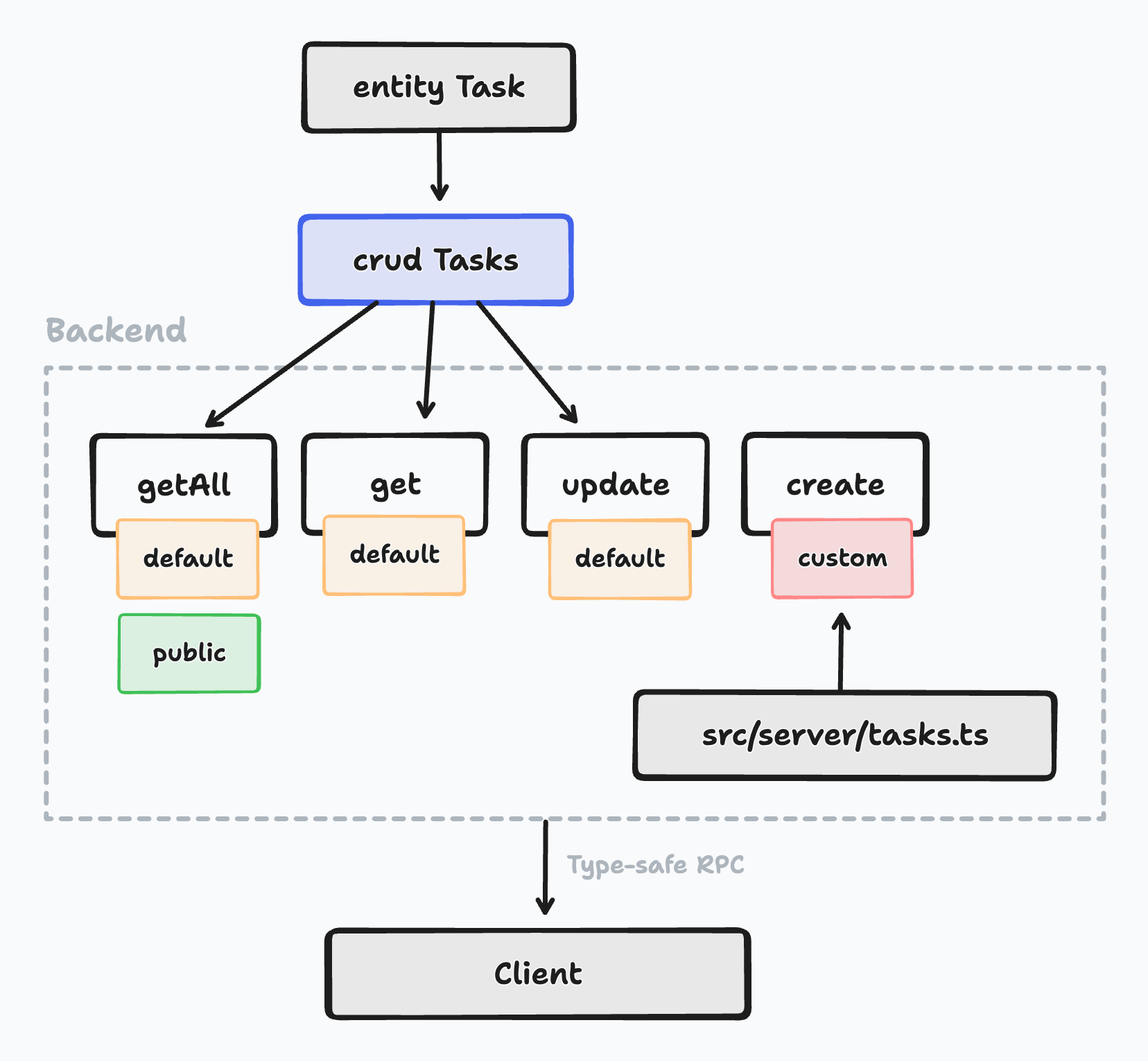
Visualization of the Tasks crud declaration
We can now use the CRUD queries and actions we just specified in our client code.
Keep reading for an example of Automatic CRUD in action, or skip ahead for the [API Reference](#api-reference)
## Example: A Simple TODO App[](#example-a-simple-todo-app "Direct link to Example: A Simple TODO App")
Let's create a full-app example that uses automatic CRUD. We'll stick to using the `Task` entity from the previous example, but we'll add a `User` entity and enable [username and password](https://wasp-lang.dev/docs/0.11.8/auth/username-and-pass) based auth.
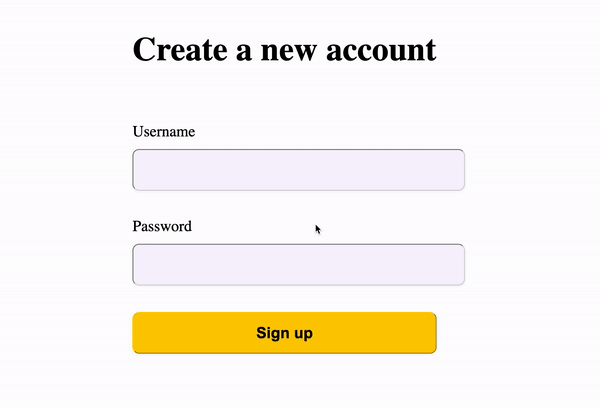
We are building a simple tasks app with username based auth
### Creating the App[](#creating-the-app "Direct link to Creating the App")
We can start by running `wasp new tasksCrudApp` and then adding the following to the `main.wasp` file:
main.wasp
```
app tasksCrudApp { wasp: { version: "^0.11.0" }, title: "Tasks Crud App", // We enabled auth and set the auth method to username and password auth: { userEntity: User, methods: { usernameAndPassword: {}, }, onAuthFailedRedirectTo: "/login", },}entity User {=psl id Int @id @default(autoincrement()) username String @unique password String tasks Task[]psl=}// We defined a Task entity on which we'll enable CRUD later onentity Task {=psl id Int @id @default(autoincrement()) description String isDone Boolean userId Int user User @relation(fields: [userId], references: [id])psl=}// Tasks app routesroute RootRoute { path: "/", to: MainPage }page MainPage { component: import { MainPage } from "@client/MainPage.jsx", authRequired: true,}route LoginRoute { path: "/login", to: LoginPage }page LoginPage { component: import { LoginPage } from "@client/LoginPage.jsx",}route SignupRoute { path: "/signup", to: SignupPage }page SignupPage { component: import { SignupPage } from "@client/SignupPage.jsx",}
```
We can then run `wasp db migrate-dev` to create the database and run the migrations.
### Adding CRUD to the `Task` Entity ✨[](#adding-crud-to-the-task-entity- "Direct link to adding-crud-to-the-task-entity-")
Let's add the following `crud` declaration to our `main.wasp` file:
main.wasp
```
// ...crud Tasks { entity: Task, operations: { getAll: {}, create: { overrideFn: import { createTask } from "@server/tasks.js", }, },}
```
You'll notice that we enabled only `getAll` and `create` operations. This means that only these operations will be available.
We also overrode the `create` operation with a custom implementation. This means that the `create` operation will not be generated, but instead, the `createTask` function from `@server/tasks.js` will be used.
### Our Custom `create` Operation[](#our-custom-create-operation "Direct link to our-custom-create-operation")
Here's the `src/server/tasks.ts` file:
* JavaScript
* TypeScript
src/server/tasks.js
```
import HttpError from '@wasp/core/HttpError.js'export const createTask = async (args, context) => { if (!context.user) { throw new HttpError(401, 'User not authenticated.') } const { description, isDone } = args const { Task } = context.entities return await Task.create({ data: { description, isDone, // Connect the task to the user that is creating it user: { connect: { id: context.user.id, }, }, }, })}
```
We made a custom `create` operation because we want to make sure that the task is connected to the user that is creating it. Automatic CRUD doesn't support this by default (yet!). Read more about the default implementations [here](#declaring-a-crud-with-default-options).
### Using the Generated CRUD Operations on the Client[](#using-the-generated-crud-operations-on-the-client "Direct link to Using the Generated CRUD Operations on the Client")
And let's use the generated operations in our client code:
* JavaScript
* TypeScript
pages/MainPage.jsx
```
import { Tasks } from '@wasp/crud/Tasks'import { useState } from 'react'export const MainPage = () => { const { data: tasks, isLoading, error } = Tasks.getAll.useQuery() const createTask = Tasks.create.useAction() const [taskDescription, setTaskDescription] = useState('') function handleCreateTask() { createTask({ description: taskDescription, isDone: false }) setTaskDescription('') } if (isLoading) return <div>Loading...</div> if (error) return <div>Error: {error.message}</div> return ( <div style={{ fontSize: '1.5rem', display: 'grid', placeContent: 'center', height: '100vh', }} > <div> <input value={taskDescription} onChange={(e) => setTaskDescription(e.target.value)} /> <button onClick={handleCreateTask}>Create task</button> </div> <ul> {tasks.map((task) => ( <li key={task.id}>{task.description}</li> ))} </ul> </div> )}
```
And here are the login and signup pages, where we are using Wasp's [Auth UI](https://wasp-lang.dev/docs/0.11.8/auth/ui) components:
* JavaScript
* TypeScript
src/client/LoginPage.jsx
```
import { LoginForm } from '@wasp/auth/forms/Login'import { Link } from 'react-router-dom'export function LoginPage() { return ( <div style={{ display: 'grid', placeContent: 'center', }} > <LoginForm /> <div> <Link to="/signup">Create an account</Link> </div> </div> )}
```
* JavaScript
* TypeScript
src/client/SignupPage.jsx
```
import { SignupForm } from '@wasp/auth/forms/Signup'export function SignupPage() { return ( <div style={{ display: 'grid', placeContent: 'center', }} > <SignupForm /> </div> )}
```
That's it. You can now run `wasp start` and see the app in action. ⚡️
You should see a login page and a signup page. After you log in, you should see a page with a list of tasks and a form to create new tasks.
## Future of CRUD Operations in Wasp[](#future-of-crud-operations-in-wasp "Direct link to Future of CRUD Operations in Wasp")
CRUD operations currently have a limited set of knowledge about the business logic they are implementing.
* For example, they don't know that a task should be connected to the user that is creating it. This is why we had to override the `create` operation in the example above.
* Another thing: they are not aware of the authorization rules. For example, they don't know that a user should not be able to create a task for another user. In the future, we will be adding role-based authorization to Wasp, and we plan to make CRUD operations aware of the authorization rules.
* Another issue is input validation and sanitization. For example, we might want to make sure that the task description is not empty.
CRUD operations are a mechanism for getting a backend up and running quickly, but it depends on the information it can get from the Wasp app. The more information that it can pick up from your app, the more powerful it will be out of the box.
We plan on supporting CRUD operations and growing them to become the easiest way to create your backend. Follow along on [this GitHub issue](https://github.com/wasp-lang/wasp/issues/1253) to see how we are doing.
## API Reference[](#api-reference "Direct link to API Reference")
CRUD declaration work on top of existing entity declaration. We'll fully explore the API using two examples:
1. A basic CRUD declaration that relies on default options.
2. A more involved CRUD declaration that uses extra options and overrides.
### Declaring a CRUD With Default Options[](#declaring-a-crud-with-default-options "Direct link to Declaring a CRUD With Default Options")
If we create CRUD operations for an entity named `Task`, like this:
* JavaScript
* TypeScript
main.wasp
```
crud Tasks { // crud name here is "Tasks" entity: Task, operations: { get: {}, getAll: {}, create: {}, update: {}, delete: {}, },}
```
Wasp will give you the following default implementations:
**get** - returns one entity based on the `id` field
```
// ...// Wasp uses the field marked with `@id` in Prisma schema as the id field.return Task.findUnique({ where: { id: args.id } })
```
**getAll** - returns all entities
```
// ...// If the operation is not public, Wasp checks if an authenticated user// is making the request.return Task.findMany()
```
**create** - creates a new entity
```
// ...return Task.create({ data: args.data })
```
**update** - updates an existing entity
```
// ...// Wasp uses the field marked with `@id` in Prisma schema as the id field.return Task.update({ where: { id: args.id }, data: args.data })
```
**delete** - deletes an existing entity
```
// ...// Wasp uses the field marked with `@id` in Prisma schema as the id field.return Task.delete({ where: { id: args.id } })
```
Current Limitations
In the default `create` and `update` implementations, we are saving all of the data that the client sends to the server. This is not always desirable, i.e. in the case when the client should not be able to modify all of the data in the entity.
[In the future](#future-of-crud-operations-in-wasp), we are planning to add validation of action input, where only the data that the user is allowed to change will be saved.
For now, the solution is to provide an override function. You can override the default implementation by using the `overrideFn` option and implementing the validation logic yourself.
### Declaring a CRUD With All Available Options[](#declaring-a-crud-with-all-available-options "Direct link to Declaring a CRUD With All Available Options")
Here's an example of a more complex CRUD declaration:
* JavaScript
* TypeScript
main.wasp
```
crud Tasks { // crud name here is "Tasks" entity: Task, operations: { getAll: { isPublic: true, // optional, defaults to false }, get: {}, create: { overrideFn: import { createTask } from "@server/tasks.js", // optional }, update: {}, },}
```
The CRUD declaration features the following fields:
* `entity: Entity` required
The entity to which the CRUD operations will be applied.
* `operations: { [operationName]: CrudOperationOptions }` required
The operations to be generated. The key is the name of the operation, and the value is the operation configuration.
* The possible values for `operationName` are:
* `getAll`
* `get`
* `create`
* `update`
* `delete`
* `CrudOperationOptions` can have the following fields:
* `isPublic: bool` - Whether the operation is public or not. If it is public, no auth is required to access it. If it is not public, it will be available only to authenticated users. Defaults to `false`.
* `overrideFn: ServerImport` - The import statement of the optional override implementation in Node.js.
#### Defining the overrides[](#defining-the-overrides "Direct link to Defining the overrides")
Like with actions and queries, you can define the implementation in a Javascript/Typescript file. The overrides are functions that take the following arguments:
* `args`
The arguments of the operation i.e. the data sent from the client.
* `context`
Context contains the `user` making the request and the `entities` object with the entity that's being operated on.
For a usage example, check the [example guide](https://wasp-lang.dev/docs/0.11.8/data-model/crud#adding-crud-to-the-task-entity-).
#### Using the CRUD operations in client code[](#using-the-crud-operations-in-client-code "Direct link to Using the CRUD operations in client code")
On the client, you import the CRUD operations from `@wasp/crud/{crud name}`. The names of the imports are the same as the names of the operations. For example, if you have a CRUD called `Tasks`, you would import the operations like this:
* JavaScript
* TypeScript
SomePage.jsx
```
import { Tasks } from '@wasp/crud/Tasks'
```
You can then access the operations like this:
* JavaScript
* TypeScript
SomePage.jsx
```
const { data } = Tasks.getAll.useQuery()const { data } = Tasks.get.useQuery({ id: 1 })const createAction = Tasks.create.useAction()const updateAction = Tasks.update.useAction()const deleteAction = Tasks.delete.useAction()
```
All CRUD operations are implemented with [Queries and Actions](https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview) under the hood, which means they come with all the features you'd expect (e.g., automatic SuperJSON serialization, full-stack type safety when using TypeScript)
* * *
Join our **community** on [Discord](https://discord.com/invite/rzdnErX), where we chat about full-stack web stuff. Join us to see what we are up to, share your opinions or get help with CRUD operations. | null | https://wasp-lang.dev/docs/0.11.8/data-model/crud | If you have a lot of experience writing full-stack apps, you probably ended up doing some of the same things many times: listing data, adding data, editing it, and deleting it. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecfbf2c4507b0-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:52 GMT | Tue, 23 Apr 2024 15:25:52 GMT | Thu, 18 Apr 2024 15:50:27 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=LXNACLQlGANUGFI5ta2vLQLrCw%2BQ9FNWix1IRjwb6HQ8ftJHpFxSsf%2B5ddE9gqsyYBPciaII0NYnnrGB7h8simiP3cxGc1hv7BYuYQLku1AvD%2FMME%2FnZZFVwa3AjIqt0"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | d800eae05344c4300be79bbe4bda1cef5c6b3ab8 | null | A71A:3F9EB3:3A3F7AF:45454E8:6627D0A7 | null | MISS | cache-iad-kiad7000111-IAD | S1713885353.855167,VS0,VE21 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/data-model/crud | og:url | Automatic CRUD | Wasp | og:title | If you have a lot of experience writing full-stack apps, you probably ended up doing some of the same things many times: listing data, adding data, editing it, and deleting it. | og:description | Automatic CRUD | Wasp | null | Automatic CRUD
If you have a lot of experience writing full-stack apps, you probably ended up doing some of the same things many times: listing data, adding data, editing it, and deleting it.
Wasp makes handling these boring bits easy by offering a higher-level concept called Automatic CRUD.
With a single declaration, you can tell Wasp to automatically generate server-side logic (i.e., Queries and Actions) for creating, reading, updating and deleting Entities. As you update definitions for your Entities, Wasp automatically regenerates the backend logic.
Early preview
This feature is currently in early preview and we are actively working on it. Read more about our plans for CRUD operations.
Overview
Imagine we have a Task entity and we want to enable CRUD operations for it.
main.wasp
entity Task {=psl
id Int @id @default(autoincrement())
description String
isDone Boolean
psl=}
We can then define a new crud called Tasks.
We specify to use the Task entity and we enable the getAll, get, create and update operations (let's say we don't need the delete operation).
main.wasp
crud Tasks {
entity: Task,
operations: {
getAll: {
isPublic: true, // by default only logged in users can perform operations
},
get: {},
create: {
overrideFn: import { createTask } from "@server/tasks.js",
},
update: {},
},
}
It uses default implementation for getAll, get, and update,
... while specifying a custom implementation for create.
getAll will be public (no auth needed), while the rest of the operations will be private.
Here's what it looks like when visualized:
Visualization of the Tasks crud declaration
We can now use the CRUD queries and actions we just specified in our client code.
Keep reading for an example of Automatic CRUD in action, or skip ahead for the API Reference
Example: A Simple TODO App
Let's create a full-app example that uses automatic CRUD. We'll stick to using the Task entity from the previous example, but we'll add a User entity and enable username and password based auth.
We are building a simple tasks app with username based auth
Creating the App
We can start by running wasp new tasksCrudApp and then adding the following to the main.wasp file:
main.wasp
app tasksCrudApp {
wasp: {
version: "^0.11.0"
},
title: "Tasks Crud App",
// We enabled auth and set the auth method to username and password
auth: {
userEntity: User,
methods: {
usernameAndPassword: {},
},
onAuthFailedRedirectTo: "/login",
},
}
entity User {=psl
id Int @id @default(autoincrement())
username String @unique
password String
tasks Task[]
psl=}
// We defined a Task entity on which we'll enable CRUD later on
entity Task {=psl
id Int @id @default(autoincrement())
description String
isDone Boolean
userId Int
user User @relation(fields: [userId], references: [id])
psl=}
// Tasks app routes
route RootRoute { path: "/", to: MainPage }
page MainPage {
component: import { MainPage } from "@client/MainPage.jsx",
authRequired: true,
}
route LoginRoute { path: "/login", to: LoginPage }
page LoginPage {
component: import { LoginPage } from "@client/LoginPage.jsx",
}
route SignupRoute { path: "/signup", to: SignupPage }
page SignupPage {
component: import { SignupPage } from "@client/SignupPage.jsx",
}
We can then run wasp db migrate-dev to create the database and run the migrations.
Adding CRUD to the Task Entity ✨
Let's add the following crud declaration to our main.wasp file:
main.wasp
// ...
crud Tasks {
entity: Task,
operations: {
getAll: {},
create: {
overrideFn: import { createTask } from "@server/tasks.js",
},
},
}
You'll notice that we enabled only getAll and create operations. This means that only these operations will be available.
We also overrode the create operation with a custom implementation. This means that the create operation will not be generated, but instead, the createTask function from @server/tasks.js will be used.
Our Custom create Operation
Here's the src/server/tasks.ts file:
JavaScript
TypeScript
src/server/tasks.js
import HttpError from '@wasp/core/HttpError.js'
export const createTask = async (args, context) => {
if (!context.user) {
throw new HttpError(401, 'User not authenticated.')
}
const { description, isDone } = args
const { Task } = context.entities
return await Task.create({
data: {
description,
isDone,
// Connect the task to the user that is creating it
user: {
connect: {
id: context.user.id,
},
},
},
})
}
We made a custom create operation because we want to make sure that the task is connected to the user that is creating it. Automatic CRUD doesn't support this by default (yet!). Read more about the default implementations here.
Using the Generated CRUD Operations on the Client
And let's use the generated operations in our client code:
JavaScript
TypeScript
pages/MainPage.jsx
import { Tasks } from '@wasp/crud/Tasks'
import { useState } from 'react'
export const MainPage = () => {
const { data: tasks, isLoading, error } = Tasks.getAll.useQuery()
const createTask = Tasks.create.useAction()
const [taskDescription, setTaskDescription] = useState('')
function handleCreateTask() {
createTask({ description: taskDescription, isDone: false })
setTaskDescription('')
}
if (isLoading) return <div>Loading...</div>
if (error) return <div>Error: {error.message}</div>
return (
<div
style={{
fontSize: '1.5rem',
display: 'grid',
placeContent: 'center',
height: '100vh',
}}
>
<div>
<input
value={taskDescription}
onChange={(e) => setTaskDescription(e.target.value)}
/>
<button onClick={handleCreateTask}>Create task</button>
</div>
<ul>
{tasks.map((task) => (
<li key={task.id}>{task.description}</li>
))}
</ul>
</div>
)
}
And here are the login and signup pages, where we are using Wasp's Auth UI components:
JavaScript
TypeScript
src/client/LoginPage.jsx
import { LoginForm } from '@wasp/auth/forms/Login'
import { Link } from 'react-router-dom'
export function LoginPage() {
return (
<div
style={{
display: 'grid',
placeContent: 'center',
}}
>
<LoginForm />
<div>
<Link to="/signup">Create an account</Link>
</div>
</div>
)
}
JavaScript
TypeScript
src/client/SignupPage.jsx
import { SignupForm } from '@wasp/auth/forms/Signup'
export function SignupPage() {
return (
<div
style={{
display: 'grid',
placeContent: 'center',
}}
>
<SignupForm />
</div>
)
}
That's it. You can now run wasp start and see the app in action. ⚡️
You should see a login page and a signup page. After you log in, you should see a page with a list of tasks and a form to create new tasks.
Future of CRUD Operations in Wasp
CRUD operations currently have a limited set of knowledge about the business logic they are implementing.
For example, they don't know that a task should be connected to the user that is creating it. This is why we had to override the create operation in the example above.
Another thing: they are not aware of the authorization rules. For example, they don't know that a user should not be able to create a task for another user. In the future, we will be adding role-based authorization to Wasp, and we plan to make CRUD operations aware of the authorization rules.
Another issue is input validation and sanitization. For example, we might want to make sure that the task description is not empty.
CRUD operations are a mechanism for getting a backend up and running quickly, but it depends on the information it can get from the Wasp app. The more information that it can pick up from your app, the more powerful it will be out of the box.
We plan on supporting CRUD operations and growing them to become the easiest way to create your backend. Follow along on this GitHub issue to see how we are doing.
API Reference
CRUD declaration work on top of existing entity declaration. We'll fully explore the API using two examples:
A basic CRUD declaration that relies on default options.
A more involved CRUD declaration that uses extra options and overrides.
Declaring a CRUD With Default Options
If we create CRUD operations for an entity named Task, like this:
JavaScript
TypeScript
main.wasp
crud Tasks { // crud name here is "Tasks"
entity: Task,
operations: {
get: {},
getAll: {},
create: {},
update: {},
delete: {},
},
}
Wasp will give you the following default implementations:
get - returns one entity based on the id field
// ...
// Wasp uses the field marked with `@id` in Prisma schema as the id field.
return Task.findUnique({ where: { id: args.id } })
getAll - returns all entities
// ...
// If the operation is not public, Wasp checks if an authenticated user
// is making the request.
return Task.findMany()
create - creates a new entity
// ...
return Task.create({ data: args.data })
update - updates an existing entity
// ...
// Wasp uses the field marked with `@id` in Prisma schema as the id field.
return Task.update({ where: { id: args.id }, data: args.data })
delete - deletes an existing entity
// ...
// Wasp uses the field marked with `@id` in Prisma schema as the id field.
return Task.delete({ where: { id: args.id } })
Current Limitations
In the default create and update implementations, we are saving all of the data that the client sends to the server. This is not always desirable, i.e. in the case when the client should not be able to modify all of the data in the entity.
In the future, we are planning to add validation of action input, where only the data that the user is allowed to change will be saved.
For now, the solution is to provide an override function. You can override the default implementation by using the overrideFn option and implementing the validation logic yourself.
Declaring a CRUD With All Available Options
Here's an example of a more complex CRUD declaration:
JavaScript
TypeScript
main.wasp
crud Tasks { // crud name here is "Tasks"
entity: Task,
operations: {
getAll: {
isPublic: true, // optional, defaults to false
},
get: {},
create: {
overrideFn: import { createTask } from "@server/tasks.js", // optional
},
update: {},
},
}
The CRUD declaration features the following fields:
entity: Entity required
The entity to which the CRUD operations will be applied.
operations: { [operationName]: CrudOperationOptions } required
The operations to be generated. The key is the name of the operation, and the value is the operation configuration.
The possible values for operationName are:
getAll
get
create
update
delete
CrudOperationOptions can have the following fields:
isPublic: bool - Whether the operation is public or not. If it is public, no auth is required to access it. If it is not public, it will be available only to authenticated users. Defaults to false.
overrideFn: ServerImport - The import statement of the optional override implementation in Node.js.
Defining the overrides
Like with actions and queries, you can define the implementation in a Javascript/Typescript file. The overrides are functions that take the following arguments:
args
The arguments of the operation i.e. the data sent from the client.
context
Context contains the user making the request and the entities object with the entity that's being operated on.
For a usage example, check the example guide.
Using the CRUD operations in client code
On the client, you import the CRUD operations from @wasp/crud/{crud name}. The names of the imports are the same as the names of the operations. For example, if you have a CRUD called Tasks, you would import the operations like this:
JavaScript
TypeScript
SomePage.jsx
import { Tasks } from '@wasp/crud/Tasks'
You can then access the operations like this:
JavaScript
TypeScript
SomePage.jsx
const { data } = Tasks.getAll.useQuery()
const { data } = Tasks.get.useQuery({ id: 1 })
const createAction = Tasks.create.useAction()
const updateAction = Tasks.update.useAction()
const deleteAction = Tasks.delete.useAction()
All CRUD operations are implemented with Queries and Actions under the hood, which means they come with all the features you'd expect (e.g., automatic SuperJSON serialization, full-stack type safety when using TypeScript)
Join our community on Discord, where we chat about full-stack web stuff. Join us to see what we are up to, share your opinions or get help with CRUD operations. | https://wasp-lang.dev/docs/0.11.8/data-model/crud |
|
2 | null | 2024-04-23T15:15:53.120Z | https://wasp-lang.dev/docs/0.11.8/data-model/operations/actions | https://wasp-lang.dev/docs/0.11.8 | http | ## Actions
We'll explain what Actions are and how to use them. If you're looking for a detailed API specification, skip ahead to the [API Reference](#api-reference).
Actions are quite similar to [Queries](https://wasp-lang.dev/docs/0.11.8/data-model/operations/queries), but with a key distinction: Actions are designed to modify and add data, while Queries are solely for reading data. Examples of Actions include adding a comment to a blog post, liking a video, or updating a product's price.
Actions and Queries work together to keep data caches up-to-date.
tip
Actions are almost identical to Queries in terms of their API. Therefore, if you're already familiar with Queries, you might find reading the entire guide repetitive.
We instead recommend skipping ahead and only reading [the differences between Queries and Actions](#differences-between-queries-and-actions), and consulting the [API Reference](#api-reference) as needed.
## Working with Actions[](#working-with-actions "Direct link to Working with Actions")
Actions are declared in Wasp and implemented in NodeJS. Wasp runs Actions within the server's context, but it also generates code that allows you to call them from anywhere in your code (either client or server) using the same interface.
This means you don't have to worry about building an HTTP API for the Action, managing server-side request handling, or even dealing with client-side response handling and caching. Instead, just focus on developing the business logic inside your Action, and let Wasp handle the rest!
To create an Action, you need to:
1. Declare the Action in Wasp using the `action` declaration.
2. Implement the Action's NodeJS functionality.
Once these two steps are completed, you can use the Action from anywhere in your code.
### Declaring Actions[](#declaring-actions "Direct link to Declaring Actions")
To create an Action in Wasp, we begin with an `action` declaration. Let's declare two Actions - one for creating a task, and another for marking tasks as done:
* JavaScript
* TypeScript
main.wasp
```
// ...action createTask { fn: import { createTask } from "@server/actions.js"}action markTaskAsDone { fn: import { markTaskAsDone } from "@server/actions.js"}
```
If you want to know about all supported options for the `action` declaration, take a look at the [API Reference](#api-reference).
The names of Wasp Actions and their implementations don't necessarily have to match. However, to avoid confusion, we'll keep them the same.
tip
Wasp uses [superjson](https://github.com/blitz-js/superjson) under the hood. This means you're not limited to only sending and receiving JSON payloads.
You can send and receive any superjson-compatible payload (like Dates, Sets, Lists, circular references, etc.) and let Wasp handle the (de)serialization.
After declaring a Wasp Action, two important things happen:
* Wasp **generates a server-side NodeJS function** that shares its name with the Action.
* Wasp **generates a client-side JavaScript function** that shares its name with the Action (e.g., `markTaskAsDone`). This function takes a single optional argument - an object containing any serializable data you wish to use inside the Action. Wasp will send this object over the network and pass it into the Action's implementation as its first positional argument (more on this when we look at the implementations). Such an abstraction works thanks to an HTTP API route handler Wasp generates on the server, which calls the Action's NodeJS implementation under the hood.
Generating these two functions ensures a uniform calling interface across the entire app (both client and server).
### Implementing Actions in Node[](#implementing-actions-in-node "Direct link to Implementing Actions in Node")
Now that we've declared the Action, what remains is to implement it. We've instructed Wasp to look for the Actions' implementations in the file `src/server/actions.ts`, so that's where we should export them from.
Here's how you might implement the previously declared Actions `createTask` and `markTaskAsDone`:
* JavaScript
* TypeScript
src/server/actions.js
```
// our "database"let nextId = 4const tasks = [ { id: 1, description: 'Buy some eggs', isDone: true }, { id: 2, description: 'Make an omelette', isDone: false }, { id: 3, description: 'Eat breakfast', isDone: false },]// You don't need to use the arguments if you don't need themexport const createTask = (args) => { const newTask = { id: nextId, isDone: false, description: args.description, } nextId += 1 tasks.push(newTask) return newTask}// The 'args' object is something sent by the caller (most often from the client)export const markTaskAsDone = (args) => { const task = tasks.find((task) => task.id === args.id) if (!task) { // We'll show how to properly handle such errors later return } task.isDone = true}
```
tip
Wasp uses [superjson](https://github.com/blitz-js/superjson) under the hood. This means you're not limited to only sending and receiving JSON payloads.
You can send and receive any superjson-compatible payload (like Dates, Sets, Lists, circular references, etc.) and let Wasp handle the (de)serialization.
For a detailed explanation of the Action definition API (i.e., arguments and return values), check the [API Reference](#api-reference).
### Using Actions[](#using-actions "Direct link to Using Actions")
To use an Action, you can import it from `@wasp` and call it directly. As mentioned, the usage doesn't change depending on whether you're on the server or the client:
* JavaScript
* TypeScript
```
import createTask from '@wasp/actions/createTask.js'import markTasAsDone from '@wasp/actions/markTasAsDone.js'// ...const newTask = await createTask({ description: 'Learn TypeScript' })await markTasAsDone({ id: 1 })
```
When using Actions on the client, you'll most likely want to use them inside a component:
* JavaScript
* TypeScript
src/client/pages/Task.jsx
```
import React from 'react'import { useQuery } from '@wasp/queries'import getTask from '@wasp/queries/getTask'import markTaskAsDone from '@wasp/actions/markTaskAsDone'export const TaskPage = ({ id }) => { const { data: task } = useQuery(getTask, { id }) if (!task) { return <h1>"Loading"</h1> } const { description, isDone } = task return ( <div> <p> <strong>Description: </strong> {description} </p> <p> <strong>Is done: </strong> {isDone ? 'Yes' : 'No'} </p> {isDone || ( <button onClick={() => markTaskAsDone({ id })}>Mark as done.</button> )} </div> )}
```
Since Actions don't require reactivity, they are safe to use inside components without a hook. Still, Wasp provides comes with the `useAction` hook you can use to enhance actions. Read all about it in the [API Reference](#api-reference).
### Error Handling[](#error-handling "Direct link to Error Handling")
For security reasons, all exceptions thrown in the Action's NodeJS implementation are sent to the client as responses with the HTTP status code `500`, with all other details removed. Hiding error details by default helps against accidentally leaking possibly sensitive information over the network.
If you do want to pass additional error information to the client, you can construct and throw an appropriate `HttpError` in your implementation:
* JavaScript
* TypeScript
src/server/actions.js
```
import HttpError from '@wasp/core/HttpError.js'export const createTask = async (args, context) => { throw new HttpError( 403, // status code "You can't do this!", // message { foo: 'bar' } // data )}
```
### Using Entities in Actions[](#using-entities-in-actions "Direct link to Using Entities in Actions")
In most cases, resources used in Actions will be [Entities](https://wasp-lang.dev/docs/0.11.8/data-model/entities). To use an Entity in your Action, add it to the `action` declaration in Wasp:
* JavaScript
* TypeScript
main.wasp
```
action createTask { fn: import { createTask } from "@server/actions.js", entities: [Task]}action markTaskAsDone { fn: import { markTaskAsDone } from "@server/actions.js", entities: [Task]}
```
Wasp will inject the specified Entity into the Action's `context` argument, giving you access to the Entity's Prisma API. Wasp invalidates frontend Query caches by looking at the Entities used by each Action/Query. Read more about Wasp's smart cache invalidation [here](#cache-invalidation).
* JavaScript
* TypeScript
src/server/actions.js
```
// The 'args' object is the payload sent by the caller (most often from the client)export const createTask = async (args, context) => { const newTask = await context.entities.Task.create({ data: { description: args.description, isDone: false, }, }) return newTask}export const markTaskAsDone = async (args, context) => { await context.entities.Task.update({ where: { id: args.id }, data: { isDone: true }, })}
```
The object `context.entities.Task` exposes `prisma.task` from [Prisma's CRUD API](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-client/crud).
### Prisma Error Helpers[](#prisma-error-helpers "Direct link to Prisma Error Helpers")
In your Operations, you may wish to handle general Prisma errors with HTTP-friendly responses.
Wasp exposes two helper functions, `isPrismaError`, and `prismaErrorToHttpError`, for this purpose. As of now, we convert two specific Prisma errors (which we will continue to expand), with the rest being `500`. See the [source here](https://github.com/wasp-lang/wasp/blob/main/waspc/e2e-test/test-outputs/waspMigrate-golden/waspMigrate/.wasp/out/server/src/utils.js).
Here's how you can import and use them:
* JavaScript
* TypeScript
```
import { isPrismaError, prismaErrorToHttpError } from "@wasp/utils.js";// ...try { await context.entities.Task.create({...})} catch (e) { if (isPrismaError(e)) { throw prismaErrorToHttpError(e) } else { throw e }}
```
## Cache Invalidation[](#cache-invalidation "Direct link to Cache Invalidation")
One of the trickiest parts of managing a web app's state is making sure the data returned by the Queries is up to date. Since Wasp uses _react-query_ for Query management, we must make sure to invalidate Queries (more specifically, their cached results managed by _react-query_) whenever they become stale.
It's possible to invalidate the caches manually through several mechanisms _react-query_ provides (e.g., refetch, direct invalidation). However, since manual cache invalidation quickly becomes complex and error-prone, Wasp offers a faster and a more effective solution to get you started: **automatic Entity-based Query cache invalidation**. Because Actions can (and most often do) modify the state while Queries read it, Wasp invalidates a Query's cache whenever an Action that uses the same Entity is executed.
For example, if the Action `createTask` and Query `getTasks` both use the Entity `Task`, executing `createTask` may cause the cached result of `getTasks` to become outdated. In response, Wasp will invalidate it, causing `getTasks` to refetch data from the server and update it.
In practice, this means that Wasp keeps the Queries "fresh" without requiring you to think about cache invalidation.
On the other hand, this kind of automatic cache invalidation can become wasteful (some updates might not be necessary) and will only work for Entities. If that's an issue, you can use the mechanisms provided by _react-query_ for now, and expect more direct support in Wasp for handling those use cases in a nice, elegant way.
If you wish to optimistically set cache values after performing an Action, you can do so using [optimistic updates](https://stackoverflow.com/a/33009713). Configure them using Wasp's [useAction hook](#the-useaction-hook-and-optimistic-updates). This is currently the only manual cache invalidation mechanism Wasps supports natively. For everything else, you can always rely on _react-query_.
## Differences Between Queries and Actions[](#differences-between-queries-and-actions "Direct link to Differences Between Queries and Actions")
Actions and Queries are two closely related concepts in Wasp. They might seem to perform similar tasks, but Wasp treats them differently, and each concept represents a different thing.
Here are the key differences between Queries and Actions:
1. Actions can (and often should) modify the server's state, while Queries are only permitted to read it. Wasp relies on you adhering to this convention when performing cache invalidations, so it's crucial to follow it.
2. Actions don't need to be reactive, so you can call them directly. However, Wasp does provide a [`useAction` React hook](#the-useaction-hook-and-optimistic-updates) for adding extra behavior to the Action (like optimistic updates).
3. `action` declarations in Wasp are mostly identical to `query` declarations. The only difference lies in the declaration's name.
## API Reference[](#api-reference "Direct link to API Reference")
### Declaring Actions in Wasp[](#declaring-actions-in-wasp "Direct link to Declaring Actions in Wasp")
The `action` declaration supports the following fields:
* `fn: ServerImport` required
The import statement of the Action's NodeJs implementation.
* `entities: [Entity]`
A list of entities you wish to use inside your Action. For instructions on using Entities in Actions, take a look at [the guide](#using-entities-in-actions).
#### Example[](#example "Direct link to Example")
* JavaScript
* TypeScript
Declaring the Action:
```
query createFoo { fn: import { createFoo } from "@server/actions.js" entities: [Foo]}
```
Enables you to import and use it anywhere in your code (on the server or the client):
```
import createFoo from '@wasp/actions/createFoo'
```
### Implementing Actions[](#implementing-actions "Direct link to Implementing Actions")
The Action's implementation is a NodeJS function that takes two arguments (it can be an `async` function if you need to use the `await` keyword). Since both arguments are positional, you can name the parameters however you want, but we'll stick with `args` and `context`:
1. `args` (type depends on the Action)
An object containing the data **passed in when calling the Action** (e.g., filtering conditions). Check [the usage examples](#using-actions) to see how to pass this object to the Action.
2. `context` (type depends on the Action)
An additional context object **passed into the Action by Wasp**. This object contains user session information, as well as information about entities. Check the [section about using entities in Actions](#using-entities-in-actions) to see how to use the entities field on the `context` object, or the [auth section](https://wasp-lang.dev/docs/0.11.8/auth/overview#using-the-contextuser-object) to see how to use the `user` object.
#### Example[](#example-1 "Direct link to Example")
* JavaScript
* TypeScript
The following Action:
```
action createFoo { fn: import { createFoo } from "@server/actions.js" entities: [Foo]}
```
Expects to find a named export `createfoo` from the file `src/server/actions.js`
actions.js
```
export const createFoo = (args, context) => { // implementation}
```
### The `useAction` Hook and Optimistic Updates[](#the-useaction-hook-and-optimistic-updates "Direct link to the-useaction-hook-and-optimistic-updates")
Make sure you understand how [Queries](https://wasp-lang.dev/docs/0.11.8/data-model/operations/queries) and [Cache Invalidation](#cache-invalidation) work before reading this chapter.
When using Actions in components, you can enhance them with the help of the `useAction` hook. This hook comes bundled with Wasp, and is used for decorating Wasp Actions. In other words, the hook returns a function whose API matches the original Action while also doing something extra under the hood (depending on how you configure it).
The `useAction` hook accepts two arguments:
* `actionFn` required
The Wasp Action (i.e., the client-side Action function generated by Wasp based on a Action declaration) you wish to enhance.
* `actionOptions`
An object configuring the extra features you want to add to the given Action. While this argument is technically optional, there is no point in using the `useAction` hook without providing it (it would be the same as using the Action directly). The Action options object supports the following fields:
* `optimisticUpdates`
An array of objects where each object defines an [optimistic update](https://stackoverflow.com/a/33009713) to perform on the Query cache. To define an optimistic update, you must specify the following properties:
* `getQuerySpecifier` required
A function returning the Query specifier (i.e., a value used to address the Query you want to update). A Query specifier is an array specifying the query function and arguments. For example, to optimistically update the Query used with `useQuery(fetchFilteredTasks, {isDone: true }]`, your `getQuerySpecifier` function would have to return the array `[fetchFilteredTasks, { isDone: true}]`. Wasp will forward the argument you pass into the decorated Action to this function (i.e., you can use the properties of the added/changed item to address the Query).
* `updateQuery` required
The function used to perform the optimistic update. It should return the desired state of the cache. Wasp will call it with the following arguments:
* `item` - The argument you pass into the decorated Action.
* `oldData` - The currently cached value for the Query identified by the specifier.
caution
The `updateQuery` function must be a pure function. It must return the desired cache value identified by the `getQuerySpecifier` function and _must not_ perform any side effects.
Also, make sure you only update the Query caches affected by your Action causing the optimistic update (Wasp cannot yet verify this).
Finally, your implementation of the `updateQuery` function should work correctly regardless of the state of `oldData` (e.g., don't rely on array positioning). If you need to do something else during your optimistic update, you can directly use _react-query_'s lower-level API (read more about it [here](#advanced-usage)).
Here's an example showing how to configure the Action `markTaskAsDone` that toggles a task's `isDone` status to perform an optimistic update:
* JavaScript
* TypeScript
src/client/pages/Task.jsx
```
import React from 'react'import { useQuery } from '@wasp/queries'import { useAction } from '@wasp/actions'import getTask from '@wasp/queries/getTask'import markTaskAsDone from '@wasp/actions/markTaskAsDone'const TaskPage = ({ id }) => { const { data: task } = useQuery(getTask, { id }) const markTaskAsDoneOptimistically = useAction(markTaskAsDone, { optimisticUpdates: [ { getQuerySpecifier: ({ id }) => [getTask, { id }], updateQuery: (_payload, oldData) => ({ ...oldData, isDone: true }), }, ], }) if (!task) { return <h1>"Loading"</h1> } const { description, isDone } = task return ( <div> <p> <strong>Description: </strong> {description} </p> <p> <strong>Is done: </strong> {isDone ? 'Yes' : 'No'} </p> {isDone || ( <button onClick={() => markTaskAsDoneOptimistically({ id })}> Mark as done. </button> )} </div> )}export default TaskPage
```
#### Advanced usage[](#advanced-usage "Direct link to Advanced usage")
The `useAction` hook currently only supports specifying optimistic updates. You can expect more features in future versions of Wasp.
Wasp's optimistic update API is deliberately small and focuses exclusively on updating Query caches (as that's the most common use case). You might need an API that offers more options or a higher level of control. If that's the case, instead of using Wasp's `useAction` hook, you can use _react-query_'s `useMutation` hook and directly work with [their low-level API](https://tanstack.com/query/v4/docs/guides/optimistic-updates?from=reactQueryV3&original=https://react-query-v3.tanstack.com/guides/optimistic-updates).
If you decide to use _react-query_'s API directly, you will need access to Query cache key. Wasp internally uses this key but abstracts it from the programmer. Still, you can easily obtain it by accessing the `queryCacheKey` property on any Query:
* JavaScript
* TypeScript
```
import getTasks from '@wasp/queries/getTasks'const queryKey = getTasks.queryCacheKey
``` | null | https://wasp-lang.dev/docs/0.11.8/data-model/operations/actions | We'll explain what Actions are and how to use them. If you're looking for a detailed API specification, skip ahead to the API Reference. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecfbf491d0798-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:52 GMT | Tue, 23 Apr 2024 15:25:52 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=HBikotm550kRP%2FMOBwLl5CW75DaF2XF7WYaoRUKnOr7CHrE4%2F9370WOenmyh4YK%2BfdhJ%2FafCG88WRwVx1fESF73DFXmUS2N8SYOwWgmn3uodhIDwXeQGhQAw%2FhjXiLbq"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | fb8df8075d2a9e7208ebc37e33c8c189ac13f65c | null | B49A:1D639D:3AFC23A:460232A:6627D0A8 | null | MISS | cache-iad-kiad7000021-IAD | S1713885353.867898,VS0,VE19 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/data-model/operations/actions | og:url | Actions | Wasp | og:title | We'll explain what Actions are and how to use them. If you're looking for a detailed API specification, skip ahead to the API Reference. | og:description | Actions | Wasp | null | Actions
We'll explain what Actions are and how to use them. If you're looking for a detailed API specification, skip ahead to the API Reference.
Actions are quite similar to Queries, but with a key distinction: Actions are designed to modify and add data, while Queries are solely for reading data. Examples of Actions include adding a comment to a blog post, liking a video, or updating a product's price.
Actions and Queries work together to keep data caches up-to-date.
tip
Actions are almost identical to Queries in terms of their API. Therefore, if you're already familiar with Queries, you might find reading the entire guide repetitive.
We instead recommend skipping ahead and only reading the differences between Queries and Actions, and consulting the API Reference as needed.
Working with Actions
Actions are declared in Wasp and implemented in NodeJS. Wasp runs Actions within the server's context, but it also generates code that allows you to call them from anywhere in your code (either client or server) using the same interface.
This means you don't have to worry about building an HTTP API for the Action, managing server-side request handling, or even dealing with client-side response handling and caching. Instead, just focus on developing the business logic inside your Action, and let Wasp handle the rest!
To create an Action, you need to:
Declare the Action in Wasp using the action declaration.
Implement the Action's NodeJS functionality.
Once these two steps are completed, you can use the Action from anywhere in your code.
Declaring Actions
To create an Action in Wasp, we begin with an action declaration. Let's declare two Actions - one for creating a task, and another for marking tasks as done:
JavaScript
TypeScript
main.wasp
// ...
action createTask {
fn: import { createTask } from "@server/actions.js"
}
action markTaskAsDone {
fn: import { markTaskAsDone } from "@server/actions.js"
}
If you want to know about all supported options for the action declaration, take a look at the API Reference.
The names of Wasp Actions and their implementations don't necessarily have to match. However, to avoid confusion, we'll keep them the same.
tip
Wasp uses superjson under the hood. This means you're not limited to only sending and receiving JSON payloads.
You can send and receive any superjson-compatible payload (like Dates, Sets, Lists, circular references, etc.) and let Wasp handle the (de)serialization.
After declaring a Wasp Action, two important things happen:
Wasp generates a server-side NodeJS function that shares its name with the Action.
Wasp generates a client-side JavaScript function that shares its name with the Action (e.g., markTaskAsDone). This function takes a single optional argument - an object containing any serializable data you wish to use inside the Action. Wasp will send this object over the network and pass it into the Action's implementation as its first positional argument (more on this when we look at the implementations). Such an abstraction works thanks to an HTTP API route handler Wasp generates on the server, which calls the Action's NodeJS implementation under the hood.
Generating these two functions ensures a uniform calling interface across the entire app (both client and server).
Implementing Actions in Node
Now that we've declared the Action, what remains is to implement it. We've instructed Wasp to look for the Actions' implementations in the file src/server/actions.ts, so that's where we should export them from.
Here's how you might implement the previously declared Actions createTask and markTaskAsDone:
JavaScript
TypeScript
src/server/actions.js
// our "database"
let nextId = 4
const tasks = [
{ id: 1, description: 'Buy some eggs', isDone: true },
{ id: 2, description: 'Make an omelette', isDone: false },
{ id: 3, description: 'Eat breakfast', isDone: false },
]
// You don't need to use the arguments if you don't need them
export const createTask = (args) => {
const newTask = {
id: nextId,
isDone: false,
description: args.description,
}
nextId += 1
tasks.push(newTask)
return newTask
}
// The 'args' object is something sent by the caller (most often from the client)
export const markTaskAsDone = (args) => {
const task = tasks.find((task) => task.id === args.id)
if (!task) {
// We'll show how to properly handle such errors later
return
}
task.isDone = true
}
tip
Wasp uses superjson under the hood. This means you're not limited to only sending and receiving JSON payloads.
You can send and receive any superjson-compatible payload (like Dates, Sets, Lists, circular references, etc.) and let Wasp handle the (de)serialization.
For a detailed explanation of the Action definition API (i.e., arguments and return values), check the API Reference.
Using Actions
To use an Action, you can import it from @wasp and call it directly. As mentioned, the usage doesn't change depending on whether you're on the server or the client:
JavaScript
TypeScript
import createTask from '@wasp/actions/createTask.js'
import markTasAsDone from '@wasp/actions/markTasAsDone.js'
// ...
const newTask = await createTask({ description: 'Learn TypeScript' })
await markTasAsDone({ id: 1 })
When using Actions on the client, you'll most likely want to use them inside a component:
JavaScript
TypeScript
src/client/pages/Task.jsx
import React from 'react'
import { useQuery } from '@wasp/queries'
import getTask from '@wasp/queries/getTask'
import markTaskAsDone from '@wasp/actions/markTaskAsDone'
export const TaskPage = ({ id }) => {
const { data: task } = useQuery(getTask, { id })
if (!task) {
return <h1>"Loading"</h1>
}
const { description, isDone } = task
return (
<div>
<p>
<strong>Description: </strong>
{description}
</p>
<p>
<strong>Is done: </strong>
{isDone ? 'Yes' : 'No'}
</p>
{isDone || (
<button onClick={() => markTaskAsDone({ id })}>Mark as done.</button>
)}
</div>
)
}
Since Actions don't require reactivity, they are safe to use inside components without a hook. Still, Wasp provides comes with the useAction hook you can use to enhance actions. Read all about it in the API Reference.
Error Handling
For security reasons, all exceptions thrown in the Action's NodeJS implementation are sent to the client as responses with the HTTP status code 500, with all other details removed. Hiding error details by default helps against accidentally leaking possibly sensitive information over the network.
If you do want to pass additional error information to the client, you can construct and throw an appropriate HttpError in your implementation:
JavaScript
TypeScript
src/server/actions.js
import HttpError from '@wasp/core/HttpError.js'
export const createTask = async (args, context) => {
throw new HttpError(
403, // status code
"You can't do this!", // message
{ foo: 'bar' } // data
)
}
Using Entities in Actions
In most cases, resources used in Actions will be Entities. To use an Entity in your Action, add it to the action declaration in Wasp:
JavaScript
TypeScript
main.wasp
action createTask {
fn: import { createTask } from "@server/actions.js",
entities: [Task]
}
action markTaskAsDone {
fn: import { markTaskAsDone } from "@server/actions.js",
entities: [Task]
}
Wasp will inject the specified Entity into the Action's context argument, giving you access to the Entity's Prisma API. Wasp invalidates frontend Query caches by looking at the Entities used by each Action/Query. Read more about Wasp's smart cache invalidation here.
JavaScript
TypeScript
src/server/actions.js
// The 'args' object is the payload sent by the caller (most often from the client)
export const createTask = async (args, context) => {
const newTask = await context.entities.Task.create({
data: {
description: args.description,
isDone: false,
},
})
return newTask
}
export const markTaskAsDone = async (args, context) => {
await context.entities.Task.update({
where: { id: args.id },
data: { isDone: true },
})
}
The object context.entities.Task exposes prisma.task from Prisma's CRUD API.
Prisma Error Helpers
In your Operations, you may wish to handle general Prisma errors with HTTP-friendly responses.
Wasp exposes two helper functions, isPrismaError, and prismaErrorToHttpError, for this purpose. As of now, we convert two specific Prisma errors (which we will continue to expand), with the rest being 500. See the source here.
Here's how you can import and use them:
JavaScript
TypeScript
import { isPrismaError, prismaErrorToHttpError } from "@wasp/utils.js";
// ...
try {
await context.entities.Task.create({...})
} catch (e) {
if (isPrismaError(e)) {
throw prismaErrorToHttpError(e)
} else {
throw e
}
}
Cache Invalidation
One of the trickiest parts of managing a web app's state is making sure the data returned by the Queries is up to date. Since Wasp uses react-query for Query management, we must make sure to invalidate Queries (more specifically, their cached results managed by react-query) whenever they become stale.
It's possible to invalidate the caches manually through several mechanisms react-query provides (e.g., refetch, direct invalidation). However, since manual cache invalidation quickly becomes complex and error-prone, Wasp offers a faster and a more effective solution to get you started: automatic Entity-based Query cache invalidation. Because Actions can (and most often do) modify the state while Queries read it, Wasp invalidates a Query's cache whenever an Action that uses the same Entity is executed.
For example, if the Action createTask and Query getTasks both use the Entity Task, executing createTask may cause the cached result of getTasks to become outdated. In response, Wasp will invalidate it, causing getTasks to refetch data from the server and update it.
In practice, this means that Wasp keeps the Queries "fresh" without requiring you to think about cache invalidation.
On the other hand, this kind of automatic cache invalidation can become wasteful (some updates might not be necessary) and will only work for Entities. If that's an issue, you can use the mechanisms provided by react-query for now, and expect more direct support in Wasp for handling those use cases in a nice, elegant way.
If you wish to optimistically set cache values after performing an Action, you can do so using optimistic updates. Configure them using Wasp's useAction hook. This is currently the only manual cache invalidation mechanism Wasps supports natively. For everything else, you can always rely on react-query.
Differences Between Queries and Actions
Actions and Queries are two closely related concepts in Wasp. They might seem to perform similar tasks, but Wasp treats them differently, and each concept represents a different thing.
Here are the key differences between Queries and Actions:
Actions can (and often should) modify the server's state, while Queries are only permitted to read it. Wasp relies on you adhering to this convention when performing cache invalidations, so it's crucial to follow it.
Actions don't need to be reactive, so you can call them directly. However, Wasp does provide a useAction React hook for adding extra behavior to the Action (like optimistic updates).
action declarations in Wasp are mostly identical to query declarations. The only difference lies in the declaration's name.
API Reference
Declaring Actions in Wasp
The action declaration supports the following fields:
fn: ServerImport required
The import statement of the Action's NodeJs implementation.
entities: [Entity]
A list of entities you wish to use inside your Action. For instructions on using Entities in Actions, take a look at the guide.
Example
JavaScript
TypeScript
Declaring the Action:
query createFoo {
fn: import { createFoo } from "@server/actions.js"
entities: [Foo]
}
Enables you to import and use it anywhere in your code (on the server or the client):
import createFoo from '@wasp/actions/createFoo'
Implementing Actions
The Action's implementation is a NodeJS function that takes two arguments (it can be an async function if you need to use the await keyword). Since both arguments are positional, you can name the parameters however you want, but we'll stick with args and context:
args (type depends on the Action)
An object containing the data passed in when calling the Action (e.g., filtering conditions). Check the usage examples to see how to pass this object to the Action.
context (type depends on the Action)
An additional context object passed into the Action by Wasp. This object contains user session information, as well as information about entities. Check the section about using entities in Actions to see how to use the entities field on the context object, or the auth section to see how to use the user object.
Example
JavaScript
TypeScript
The following Action:
action createFoo {
fn: import { createFoo } from "@server/actions.js"
entities: [Foo]
}
Expects to find a named export createfoo from the file src/server/actions.js
actions.js
export const createFoo = (args, context) => {
// implementation
}
The useAction Hook and Optimistic Updates
Make sure you understand how Queries and Cache Invalidation work before reading this chapter.
When using Actions in components, you can enhance them with the help of the useAction hook. This hook comes bundled with Wasp, and is used for decorating Wasp Actions. In other words, the hook returns a function whose API matches the original Action while also doing something extra under the hood (depending on how you configure it).
The useAction hook accepts two arguments:
actionFn required
The Wasp Action (i.e., the client-side Action function generated by Wasp based on a Action declaration) you wish to enhance.
actionOptions
An object configuring the extra features you want to add to the given Action. While this argument is technically optional, there is no point in using the useAction hook without providing it (it would be the same as using the Action directly). The Action options object supports the following fields:
optimisticUpdates
An array of objects where each object defines an optimistic update to perform on the Query cache. To define an optimistic update, you must specify the following properties:
getQuerySpecifier required
A function returning the Query specifier (i.e., a value used to address the Query you want to update). A Query specifier is an array specifying the query function and arguments. For example, to optimistically update the Query used with useQuery(fetchFilteredTasks, {isDone: true }], your getQuerySpecifier function would have to return the array [fetchFilteredTasks, { isDone: true}]. Wasp will forward the argument you pass into the decorated Action to this function (i.e., you can use the properties of the added/changed item to address the Query).
updateQuery required
The function used to perform the optimistic update. It should return the desired state of the cache. Wasp will call it with the following arguments:
item - The argument you pass into the decorated Action.
oldData - The currently cached value for the Query identified by the specifier.
caution
The updateQuery function must be a pure function. It must return the desired cache value identified by the getQuerySpecifier function and must not perform any side effects.
Also, make sure you only update the Query caches affected by your Action causing the optimistic update (Wasp cannot yet verify this).
Finally, your implementation of the updateQuery function should work correctly regardless of the state of oldData (e.g., don't rely on array positioning). If you need to do something else during your optimistic update, you can directly use react-query's lower-level API (read more about it here).
Here's an example showing how to configure the Action markTaskAsDone that toggles a task's isDone status to perform an optimistic update:
JavaScript
TypeScript
src/client/pages/Task.jsx
import React from 'react'
import { useQuery } from '@wasp/queries'
import { useAction } from '@wasp/actions'
import getTask from '@wasp/queries/getTask'
import markTaskAsDone from '@wasp/actions/markTaskAsDone'
const TaskPage = ({ id }) => {
const { data: task } = useQuery(getTask, { id })
const markTaskAsDoneOptimistically = useAction(markTaskAsDone, {
optimisticUpdates: [
{
getQuerySpecifier: ({ id }) => [getTask, { id }],
updateQuery: (_payload, oldData) => ({ ...oldData, isDone: true }),
},
],
})
if (!task) {
return <h1>"Loading"</h1>
}
const { description, isDone } = task
return (
<div>
<p>
<strong>Description: </strong>
{description}
</p>
<p>
<strong>Is done: </strong>
{isDone ? 'Yes' : 'No'}
</p>
{isDone || (
<button onClick={() => markTaskAsDoneOptimistically({ id })}>
Mark as done.
</button>
)}
</div>
)
}
export default TaskPage
Advanced usage
The useAction hook currently only supports specifying optimistic updates. You can expect more features in future versions of Wasp.
Wasp's optimistic update API is deliberately small and focuses exclusively on updating Query caches (as that's the most common use case). You might need an API that offers more options or a higher level of control. If that's the case, instead of using Wasp's useAction hook, you can use react-query's useMutation hook and directly work with their low-level API.
If you decide to use react-query's API directly, you will need access to Query cache key. Wasp internally uses this key but abstracts it from the programmer. Still, you can easily obtain it by accessing the queryCacheKey property on any Query:
JavaScript
TypeScript
import getTasks from '@wasp/queries/getTasks'
const queryKey = getTasks.queryCacheKey | https://wasp-lang.dev/docs/0.11.8/data-model/operations/actions |
|
2 | null | 2024-04-23T15:15:53.034Z | https://wasp-lang.dev/docs/0.11.8/data-model/operations/queries | https://wasp-lang.dev/docs/0.11.8 | http | ## Queries
We'll explain what Queries are and how to use them. If you're looking for a detailed API specification, skip ahead to the [API Reference](#api-reference).
You can use Queries to fetch data from the server. They shouldn't modify the server's state. Fetching all comments on a blog post, a list of users that liked a video, information about a single product based on its ID... All of these are perfect use cases for a Query.
tip
Queries are fairly similar to Actions in terms of their API. Therefore, if you're already familiar with Actions, you might find reading the entire guide repetitive.
We instead recommend skipping ahead and only reading [the differences between Queries and Actions](https://wasp-lang.dev/docs/0.11.8/data-model/operations/actions#differences-between-queries-and-actions), and consulting the [API Reference](#api-reference) as needed.
## Working with Queries[](#working-with-queries "Direct link to Working with Queries")
You declare queries in the `.wasp` file and implement them using NodeJS. Wasp not only runs these queries within the server's context but also creates code that enables you to call them from any part of your codebase, whether it's on the client or server side.
This means you don't have to build an HTTP API for your query, manage server-side request handling, or even deal with client-side response handling and caching. Instead, just concentrate on implementing the business logic inside your query, and let Wasp handle the rest!
To create a Query, you must:
1. Declare the Query in Wasp using the `query` declaration.
2. Define the Query's NodeJS implementation.
After completing these two steps, you'll be able to use the Query from any point in your code.
### Declaring Queries[](#declaring-queries "Direct link to Declaring Queries")
To create a Query in Wasp, we begin with a `query` declaration.
Let's declare two Queries - one to fetch all tasks, and another to fetch tasks based on a filter, such as whether a task is done:
* JavaScript
* TypeScript
main.wasp
```
// ...query getAllTasks { fn: import { getAllTasks } from "@server/queries.js"}query getFilteredTasks { fn: import { getFilteredTasks } from "@server/queries.js"}
```
If you want to know about all supported options for the `query` declaration, take a look at the [API Reference](#api-reference).
The names of Wasp Queries and their implementations don't need to match, but we'll keep them the same to avoid confusion.
info
You might have noticed that we told Wasp to import Query implementations that don't yet exist. Don't worry about that for now. We'll write the implementations imported from `queries.ts` in the next section.
It's a good idea to start with the high-level concept (i.e., the Query declaration in the Wasp file) and only then deal with the implementation details (i.e., the Query's implementation in JavaScript).
After declaring a Wasp Query, two important things happen:
* Wasp **generates a server-side NodeJS function** that shares its name with the Query.
* Wasp **generates a client-side JavaScript function** that shares its name with the Query (e.g., `getFilteredTasks`). This function takes a single optional argument - an object containing any serializable data you wish to use inside the Query. Wasp will send this object over the network and pass it into the Query's implementation as its first positional argument (more on this when we look at the implementations). Such an abstraction works thanks to an HTTP API route handler Wasp generates on the server, which calls the Query's NodeJS implementation under the hood.
Generating these two functions ensures a uniform calling interface across the entire app (both client and server).
### Implementing Queries in Node[](#implementing-queries-in-node "Direct link to Implementing Queries in Node")
Now that we've declared the Query, what remains is to implement it. We've instructed Wasp to look for the Queries' implementations in the file `src/server/queries.ts`, so that's where we should export them from.
Here's how you might implement the previously declared Queries `getAllTasks` and `getFilteredTasks`:
* JavaScript
* TypeScript
src/server/queries.js
```
// our "database"const tasks = [ { id: 1, description: 'Buy some eggs', isDone: true }, { id: 2, description: 'Make an omelette', isDone: false }, { id: 3, description: 'Eat breakfast', isDone: false },]// You don't need to use the arguments if you don't need themexport const getAllTasks = () => { return tasks}// The 'args' object is something sent by the caller (most often from the client)export const getFilteredTasks = (args) => { const { isDone } = args return tasks.filter((task) => task.isDone === isDone)}
```
tip
Wasp uses [superjson](https://github.com/blitz-js/superjson) under the hood. This means you're not limited to only sending and receiving JSON payloads.
You can send and receive any superjson-compatible payload (like Dates, Sets, Lists, circular references, etc.) and let Wasp handle the (de)serialization.
For a detailed explanation of the Query definition API (i.e., arguments and return values), check the [API Reference](#api-reference).
### Using Queries[](#using-queries "Direct link to Using Queries")
To use a Query, you can import it from `@wasp` and call it directly. As mentioned, the usage doesn't change depending on whether you're on the server or the client:
* JavaScript
* TypeScript
```
import getAllTasks from '@wasp/queries/getAllTasks.js'import getFilteredTasks from '@wasp/queries/getFilteredTasks.js'// ...const allTasks = await getAllTasks()const doneTasks = await getFilteredTasks({ isDone: true })
```
#### The `useQuery` hook[](#the-usequery-hook "Direct link to the-usequery-hook")
When using Queries on the client, you can make them reactive with the `useQuery` hook. This hook comes bundled with Wasp and is a thin wrapper around the `useQuery` hook from [_react-query_](https://github.com/tannerlinsley/react-query). The only difference is that you don't need to supply the key - Wasp handles this for you automatically.
Here's an example of calling the Queries using the `useQuery` hook:
* JavaScript
* TypeScript
src/client/MainPage.jsx
```
import React from 'react'import { useQuery } from '@wasp/queries'import getAllTasks from '@wasp/queries/getAllTasks'import getFilteredTasks from '@wasp/queries/getFilteredTasks'const MainPage = () => { const { data: allTasks, error: error1 } = useQuery(getAllTasks) const { data: doneTasks, error: error2 } = useQuery(getFilteredTasks, { isDone: true, }) if (error1 !== null || error2 !== null) { return <div>There was an error</div> } return ( <div> <h2>All Tasks</h2> {allTasks && allTasks.length > 0 ? allTasks.map((task) => <Task key={task.id} {...task} />) : 'No tasks'} <h2>Finished Tasks</h2> {doneTasks && doneTasks.length > 0 ? doneTasks.map((task) => <Task key={task.id} {...task} />) : 'No finished tasks'} </div> )}const Task = ({ description, isDone }: Task) => { return ( <div> <p> <strong>Description: </strong> {description} </p> <p> <strong>Is done: </strong> {isDone ? 'Yes' : 'No'} </p> </div> )}export default MainPage
```
For a detailed specification of the `useQuery` hook, check the [API Reference](#api-reference).
### Error Handling[](#error-handling "Direct link to Error Handling")
For security reasons, all exceptions thrown in the Query's NodeJS implementation are sent to the client as responses with the HTTP status code `500`, with all other details removed. Hiding error details by default helps against accidentally leaking possibly sensitive information over the network.
If you do want to pass additional error information to the client, you can construct and throw an appropriate `HttpError` in your implementation:
* JavaScript
* TypeScript
src/server/queries.js
```
import HttpError from '@wasp/core/HttpError.js'export const getAllTasks = async (args, context) => { throw new HttpError( 403, // status code "You can't do this!", // message { foo: 'bar' } // data )}
```
If the status code is `4xx`, the client will receive a response object with the corresponding `message` and `data` fields, and it will rethrow the error (including these fields). To prevent information leakage, the server won't forward these fields for any other HTTP status codes.
### Using Entities in Queries[](#using-entities-in-queries "Direct link to Using Entities in Queries")
In most cases, resources used in Queries will be [Entities](https://wasp-lang.dev/docs/0.11.8/data-model/entities). To use an Entity in your Query, add it to the `query` declaration in Wasp:
* JavaScript
* TypeScript
main.wasp
```
query getAllTasks { fn: import { getAllTasks } from "@server/queries.js", entities: [Task]}query getFilteredTasks { fn: import { getFilteredTasks } from "@server/queries.js", entities: [Task]}
```
Wasp will inject the specified Entity into the Query's `context` argument, giving you access to the Entity's Prisma API:
* JavaScript
* TypeScript
src/server/queries.js
```
export const getAllTasks = async (args, context) => { return context.entities.Task.findMany({})}export const getFilteredTasks = async (args, context) => { return context.entities.Task.findMany({ where: { isDone: args.isDone }, })}
```
The object `context.entities.Task` exposes `prisma.task` from [Prisma's CRUD API](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-client/crud).
## API Reference[](#api-reference "Direct link to API Reference")
### Declaring Queries[](#declaring-queries-1 "Direct link to Declaring Queries")
The `query` declaration supports the following fields:
* `fn: ServerImport` required
The import statement of the Query's NodeJs implementation.
* `entities: [Entity]`
A list of entities you wish to use inside your Query. For instructions on using Entities in Queries, take a look at [the guide](#using-entities-in-queries).
#### Example[](#example "Direct link to Example")
* JavaScript
* TypeScript
Declaring the Query:
```
query getFoo { fn: import { getFoo } from "@server/queries.js" entities: [Foo]}
```
Enables you to import and use it anywhere in your code (on the server or the client):
```
import getFoo from '@wasp/queries/getFoo'
```
### Implementing Queries[](#implementing-queries "Direct link to Implementing Queries")
The Query's implementation is a NodeJS function that takes two arguments (it can be an `async` function if you need to use the `await` keyword). Since both arguments are positional, you can name the parameters however you want, but we'll stick with `args` and `context`:
1. `args` (type depends on the Query)
An object containing the data **passed in when calling the query** (e.g., filtering conditions). Check [the usage examples](#using-queries) to see how to pass this object to the Query.
2. `context` (type depends on the Query)
An additional context object **passed into the Query by Wasp**. This object contains user session information, as well as information about entities. Check the [section about using entities in Queries](#using-entities-in-queries) to see how to use the entities field on the `context` object, or the [auth section](https://wasp-lang.dev/docs/0.11.8/auth/overview#using-the-contextuser-object) to see how to use the `user` object.
#### Example[](#example-1 "Direct link to Example")
* JavaScript
* TypeScript
The following Query:
```
query getFoo { fn: import { getFoo } from "@server/queries.js" entities: [Foo]}
```
Expects to find a named export `getFoo` from the file `src/server/queries.js`
queries.js
```
export const getFoo = (args, context) => { // implementation}
```
### The `useQuery` Hook[](#the-usequery-hook-1 "Direct link to the-usequery-hook-1")
Wasp's `useQuery` hook is a thin wrapper around the `useQuery` hook from [_react-query_](https://github.com/tannerlinsley/react-query). One key difference is that Wasp doesn't expect you to supply the cache key - it takes care of it under the hood.
Wasp's `useQuery` hook accepts three arguments:
* `queryFn` required
The client-side query function generated by Wasp based on a `query` declaration in your `.wasp` file.
* `queryFnArgs`
The arguments object (payload) you wish to pass into the Query. The Query's NodeJS implementation will receive this object as its first positional argument.
* `options`
A _react-query_ `options` object. Use this to change [the default behavior](https://react-query.tanstack.com/guides/important-defaults) for this particular Query. If you want to change the global defaults, you can do so in the [client setup function](https://wasp-lang.dev/docs/0.11.8/project/client-config#overriding-default-behaviour-for-queries).
For an example of usage, check [this section](#the-usequery-hook). | null | https://wasp-lang.dev/docs/0.11.8/data-model/operations/queries | We'll explain what Queries are and how to use them. If you're looking for a detailed API specification, skip ahead to the API Reference. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecfbf3b1d7f72-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:15:52 GMT | Tue, 23 Apr 2024 15:25:52 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=lE%2BnkT87hXwtCuZHZ8aRWfA%2FWVe2GDhP3Wz0An65ciZNZVRP8KZgE0tQLYI3igG4lixgSCe12WWxEpv4pqJ7PqmUrro9hMMek7TJosjy4rrYla10Y%2B05F0pKXw91nKwB"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 546dae0ce1482e0ff4039642a4ad275b5cededb5 | null | AE7E:16C4F4:3911115:44175DC:6627D0A8 | HIT | MISS | cache-iad-kiad7000113-IAD | S1713885353.860800,VS0,VE30 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/data-model/operations/queries | og:url | Queries | Wasp | og:title | We'll explain what Queries are and how to use them. If you're looking for a detailed API specification, skip ahead to the API Reference. | og:description | Queries | Wasp | null | Queries
We'll explain what Queries are and how to use them. If you're looking for a detailed API specification, skip ahead to the API Reference.
You can use Queries to fetch data from the server. They shouldn't modify the server's state. Fetching all comments on a blog post, a list of users that liked a video, information about a single product based on its ID... All of these are perfect use cases for a Query.
tip
Queries are fairly similar to Actions in terms of their API. Therefore, if you're already familiar with Actions, you might find reading the entire guide repetitive.
We instead recommend skipping ahead and only reading the differences between Queries and Actions, and consulting the API Reference as needed.
Working with Queries
You declare queries in the .wasp file and implement them using NodeJS. Wasp not only runs these queries within the server's context but also creates code that enables you to call them from any part of your codebase, whether it's on the client or server side.
This means you don't have to build an HTTP API for your query, manage server-side request handling, or even deal with client-side response handling and caching. Instead, just concentrate on implementing the business logic inside your query, and let Wasp handle the rest!
To create a Query, you must:
Declare the Query in Wasp using the query declaration.
Define the Query's NodeJS implementation.
After completing these two steps, you'll be able to use the Query from any point in your code.
Declaring Queries
To create a Query in Wasp, we begin with a query declaration.
Let's declare two Queries - one to fetch all tasks, and another to fetch tasks based on a filter, such as whether a task is done:
JavaScript
TypeScript
main.wasp
// ...
query getAllTasks {
fn: import { getAllTasks } from "@server/queries.js"
}
query getFilteredTasks {
fn: import { getFilteredTasks } from "@server/queries.js"
}
If you want to know about all supported options for the query declaration, take a look at the API Reference.
The names of Wasp Queries and their implementations don't need to match, but we'll keep them the same to avoid confusion.
info
You might have noticed that we told Wasp to import Query implementations that don't yet exist. Don't worry about that for now. We'll write the implementations imported from queries.ts in the next section.
It's a good idea to start with the high-level concept (i.e., the Query declaration in the Wasp file) and only then deal with the implementation details (i.e., the Query's implementation in JavaScript).
After declaring a Wasp Query, two important things happen:
Wasp generates a server-side NodeJS function that shares its name with the Query.
Wasp generates a client-side JavaScript function that shares its name with the Query (e.g., getFilteredTasks). This function takes a single optional argument - an object containing any serializable data you wish to use inside the Query. Wasp will send this object over the network and pass it into the Query's implementation as its first positional argument (more on this when we look at the implementations). Such an abstraction works thanks to an HTTP API route handler Wasp generates on the server, which calls the Query's NodeJS implementation under the hood.
Generating these two functions ensures a uniform calling interface across the entire app (both client and server).
Implementing Queries in Node
Now that we've declared the Query, what remains is to implement it. We've instructed Wasp to look for the Queries' implementations in the file src/server/queries.ts, so that's where we should export them from.
Here's how you might implement the previously declared Queries getAllTasks and getFilteredTasks:
JavaScript
TypeScript
src/server/queries.js
// our "database"
const tasks = [
{ id: 1, description: 'Buy some eggs', isDone: true },
{ id: 2, description: 'Make an omelette', isDone: false },
{ id: 3, description: 'Eat breakfast', isDone: false },
]
// You don't need to use the arguments if you don't need them
export const getAllTasks = () => {
return tasks
}
// The 'args' object is something sent by the caller (most often from the client)
export const getFilteredTasks = (args) => {
const { isDone } = args
return tasks.filter((task) => task.isDone === isDone)
}
tip
Wasp uses superjson under the hood. This means you're not limited to only sending and receiving JSON payloads.
You can send and receive any superjson-compatible payload (like Dates, Sets, Lists, circular references, etc.) and let Wasp handle the (de)serialization.
For a detailed explanation of the Query definition API (i.e., arguments and return values), check the API Reference.
Using Queries
To use a Query, you can import it from @wasp and call it directly. As mentioned, the usage doesn't change depending on whether you're on the server or the client:
JavaScript
TypeScript
import getAllTasks from '@wasp/queries/getAllTasks.js'
import getFilteredTasks from '@wasp/queries/getFilteredTasks.js'
// ...
const allTasks = await getAllTasks()
const doneTasks = await getFilteredTasks({ isDone: true })
The useQuery hook
When using Queries on the client, you can make them reactive with the useQuery hook. This hook comes bundled with Wasp and is a thin wrapper around the useQuery hook from react-query. The only difference is that you don't need to supply the key - Wasp handles this for you automatically.
Here's an example of calling the Queries using the useQuery hook:
JavaScript
TypeScript
src/client/MainPage.jsx
import React from 'react'
import { useQuery } from '@wasp/queries'
import getAllTasks from '@wasp/queries/getAllTasks'
import getFilteredTasks from '@wasp/queries/getFilteredTasks'
const MainPage = () => {
const { data: allTasks, error: error1 } = useQuery(getAllTasks)
const { data: doneTasks, error: error2 } = useQuery(getFilteredTasks, {
isDone: true,
})
if (error1 !== null || error2 !== null) {
return <div>There was an error</div>
}
return (
<div>
<h2>All Tasks</h2>
{allTasks && allTasks.length > 0
? allTasks.map((task) => <Task key={task.id} {...task} />)
: 'No tasks'}
<h2>Finished Tasks</h2>
{doneTasks && doneTasks.length > 0
? doneTasks.map((task) => <Task key={task.id} {...task} />)
: 'No finished tasks'}
</div>
)
}
const Task = ({ description, isDone }: Task) => {
return (
<div>
<p>
<strong>Description: </strong>
{description}
</p>
<p>
<strong>Is done: </strong>
{isDone ? 'Yes' : 'No'}
</p>
</div>
)
}
export default MainPage
For a detailed specification of the useQuery hook, check the API Reference.
Error Handling
For security reasons, all exceptions thrown in the Query's NodeJS implementation are sent to the client as responses with the HTTP status code 500, with all other details removed. Hiding error details by default helps against accidentally leaking possibly sensitive information over the network.
If you do want to pass additional error information to the client, you can construct and throw an appropriate HttpError in your implementation:
JavaScript
TypeScript
src/server/queries.js
import HttpError from '@wasp/core/HttpError.js'
export const getAllTasks = async (args, context) => {
throw new HttpError(
403, // status code
"You can't do this!", // message
{ foo: 'bar' } // data
)
}
If the status code is 4xx, the client will receive a response object with the corresponding message and data fields, and it will rethrow the error (including these fields). To prevent information leakage, the server won't forward these fields for any other HTTP status codes.
Using Entities in Queries
In most cases, resources used in Queries will be Entities. To use an Entity in your Query, add it to the query declaration in Wasp:
JavaScript
TypeScript
main.wasp
query getAllTasks {
fn: import { getAllTasks } from "@server/queries.js",
entities: [Task]
}
query getFilteredTasks {
fn: import { getFilteredTasks } from "@server/queries.js",
entities: [Task]
}
Wasp will inject the specified Entity into the Query's context argument, giving you access to the Entity's Prisma API:
JavaScript
TypeScript
src/server/queries.js
export const getAllTasks = async (args, context) => {
return context.entities.Task.findMany({})
}
export const getFilteredTasks = async (args, context) => {
return context.entities.Task.findMany({
where: { isDone: args.isDone },
})
}
The object context.entities.Task exposes prisma.task from Prisma's CRUD API.
API Reference
Declaring Queries
The query declaration supports the following fields:
fn: ServerImport required
The import statement of the Query's NodeJs implementation.
entities: [Entity]
A list of entities you wish to use inside your Query. For instructions on using Entities in Queries, take a look at the guide.
Example
JavaScript
TypeScript
Declaring the Query:
query getFoo {
fn: import { getFoo } from "@server/queries.js"
entities: [Foo]
}
Enables you to import and use it anywhere in your code (on the server or the client):
import getFoo from '@wasp/queries/getFoo'
Implementing Queries
The Query's implementation is a NodeJS function that takes two arguments (it can be an async function if you need to use the await keyword). Since both arguments are positional, you can name the parameters however you want, but we'll stick with args and context:
args (type depends on the Query)
An object containing the data passed in when calling the query (e.g., filtering conditions). Check the usage examples to see how to pass this object to the Query.
context (type depends on the Query)
An additional context object passed into the Query by Wasp. This object contains user session information, as well as information about entities. Check the section about using entities in Queries to see how to use the entities field on the context object, or the auth section to see how to use the user object.
Example
JavaScript
TypeScript
The following Query:
query getFoo {
fn: import { getFoo } from "@server/queries.js"
entities: [Foo]
}
Expects to find a named export getFoo from the file src/server/queries.js
queries.js
export const getFoo = (args, context) => {
// implementation
}
The useQuery Hook
Wasp's useQuery hook is a thin wrapper around the useQuery hook from react-query. One key difference is that Wasp doesn't expect you to supply the cache key - it takes care of it under the hood.
Wasp's useQuery hook accepts three arguments:
queryFn required
The client-side query function generated by Wasp based on a query declaration in your .wasp file.
queryFnArgs
The arguments object (payload) you wish to pass into the Query. The Query's NodeJS implementation will receive this object as its first positional argument.
options
A react-query options object. Use this to change the default behavior for this particular Query. If you want to change the global defaults, you can do so in the client setup function.
For an example of usage, check this section. | https://wasp-lang.dev/docs/0.11.8/data-model/operations/queries |
|
2 | null | 2024-04-23T15:16:00.160Z | https://wasp-lang.dev/docs/0.11.8/data-model/backends | https://wasp-lang.dev/docs/0.11.8 | http | ## Databases
[Entities](https://wasp-lang.dev/docs/0.11.8/data-model/entities), [Operations](https://wasp-lang.dev/docs/0.11.8/data-model/operations/overview) and [Automatic CRUD](https://wasp-lang.dev/docs/0.11.8/data-model/crud) together make a high-level interface for working with your app's data. Still, all that data has to live somewhere, so let's see how Wasp deals with databases.
## Supported Database Backends[](#supported-database-backends "Direct link to Supported Database Backends")
Wasp supports multiple database backends. We'll list and explain each one.
### SQLite[](#sqlite "Direct link to SQLite")
The default database Wasp uses is [SQLite](https://www.sqlite.org/index.html).
SQLite is a great way for getting started with a new project because it doesn't require any configuration, but Wasp can only use it in development. Once you want to deploy your Wasp app to production, you'll need to switch to PostgreSQL and stick with it.
Fortunately, migrating from SQLite to PostgreSQL is pretty simple, and we have [a guide](#migrating-from-sqlite-to-postgresql) to help you.
### PostgreSQL[](#postgresql "Direct link to PostgreSQL")
[PostgreSQL](https://www.postgresql.org/) is the most advanced open source database and the fourth most popular database overall. It's been in active development for 20 years. Therefore, if you're looking for a battle-tested database, look no further.
To use Wasp with PostgreSQL, you'll have to ensure a database instance is running during development. Wasp needs access to your database for commands such as `wasp start` or `wasp db migrate-dev` and expects to find a connection string in the `DATABASE_URL` environment variable.
We cover all supported ways of connecting to a database in [the next section](#connecting-to-a-database).
### Migrating from SQLite to PostgreSQL[](#migrating-from-sqlite-to-postgresql "Direct link to Migrating from SQLite to PostgreSQL")
To run your Wasp app in production, you'll need to switch from SQLite to PostgreSQL.
1. Set the `app.db.system` field to PostgreSQL.
main.wasp
```
app MyApp { title: "My app", // ... db: { system: PostgreSQL, // ... }}
```
2. Delete all the old migrations, since they are SQLite migrations and can't be used with PostgreSQL, as well as the SQLite database by running [`wasp clean`](https://wasp-lang.dev/docs/general/cli#project-commands):
```
rm -r migrations/wasp clean
```
3. Ensure your new database is running (check the [section on connecing to a database](#connecting-to-a-database) to see how). Leave it running, since we need it for the next step.
4. In a different terminal, run `wasp db migrate-dev` to apply the changes and create a new initial migration.
5. That is it, you are all done!
## Connecting to a Database[](#connecting-to-a-database "Direct link to Connecting to a Database")
Assuming you're not using SQLite, Wasp offers two ways of connecting your app to a database instance:
1. A ready-made dev database that requires minimal setup and is great for quick prototyping.
2. A "real" database Wasp can connect to and use in production.
### Using the Dev Database Provided by Wasp[](#using-the-dev-database-provided-by-wasp "Direct link to Using the Dev Database Provided by Wasp")
The command `wasp start db` will start a default PostgreSQL dev database for you.
Your Wasp app will automatically connect to it, just keep `wasp start db` running in the background. Also, make sure that:
* You have [Docker installed](https://www.docker.com/get-started/) and in `PATH`.
* The port `5432` isn't taken.
### Connecting to an existing database[](#connecting-to-an-existing-database "Direct link to Connecting to an existing database")
If you want to spin up your own dev database (or connect to an external one), you can tell Wasp about it using the `DATABASE_URL` environment variable. Wasp will use the value of `DATABASE_URL` as a connection string.
The easiest way to set the necessary `DATABASE_URL` environment variable is by adding it to the [.env.server](https://wasp-lang.dev/docs/0.11.8/project/env-vars) file in the root dir of your Wasp project (if that file doesn't yet exist, create it).
Alternatively, you can set it inline when running `wasp` (this applies to all environment variables):
```
DATABASE_URL=<my-db-url> wasp ...
```
This trick is useful for running a certain `wasp` command on a specific database. For example, you could do:
```
DATABASE_URL=<production-db-url> wasp db seed myProductionSeed
```
This command seeds the data for a fresh staging or production database. To more precisely understand how seeding works, keep reading.
## Seeding the Database[](#seeding-the-database "Direct link to Seeding the Database")
**Database seeding** is a term used for populating the database with some initial data.
Seeding is most commonly used for two following scenarios:
1. To put the development database into a state convenient for working and testing.
2. To initialize any database (`dev`, `staging`, or `prod`) with essential data it requires to operate. For example, populating the Currency table with default currencies, or the Country table with all available countries.
### Writing a Seed Function[](#writing-a-seed-function "Direct link to Writing a Seed Function")
You can define as many **seed functions** as you want in an array under the `app.db.seeds` field:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { // ... db: { // ... seeds: [ import { devSeedSimple } from "@server/dbSeeds.js", import { prodSeed } from "@server/dbSeeds.js" ] }}
```
Each seed function must be an async function that takes one argument, `prismaClient`, which is a [Prisma Client](https://www.prisma.io/docs/concepts/components/prisma-client/crud) instance used to interact with the database. This is the same Prisma Client instance that Wasp uses internally and thus includes all of the usual features (e.g., password hashing).
Since a seed function falls under server-side code, it can import other server-side functions. This is convenient because you might want to seed the database using Actions.
Here's an example of a seed function that imports an Action:
* JavaScript
* TypeScript
```
import { createTask } from "./actions.js";export const devSeedSimple = async (prismaClient) => { const user = await createUser(prismaClient, { username: "RiuTheDog", password: "bark1234", }); await createTask( { description: "Chase the cat" }, { user, entities: { Task: prismaClient.task } } );};async function createUser(prismaClient, data) { const { password, ...newUser } = await prismaClient.user.create({ data }); return newUser;}
```
### Running seed functions[](#running-seed-functions "Direct link to Running seed functions")
Run the command `wasp db seed` and Wasp will ask you which seed function you'd like to run (if you've defined more than one).
Alternatively, run the command `wasp db seed <seed-name>` to choose a specific seed function right away, for example:
```
wasp db seed devSeedSimple
```
Check the [API Reference](#cli-commands-for-seeding-the-database) for more details on these commands.
tip
You'll often want to call `wasp db seed` right after you run `wasp db reset`, as it makes sense to fill the database with initial data after clearing it.
## Prisma Configuration[](#prisma-configuration "Direct link to Prisma Configuration")
Wasp uses [Prisma](https://www.prisma.io/) to interact with the database. Prisma is a "Next-generation Node.js and TypeScript ORM" that provides a type-safe API for working with your database.
### Prisma Preview Features[](#prisma-preview-features "Direct link to Prisma Preview Features")
Prisma is still in active development and some of its features are not yet stable. To use them, you have to enable them in the `app.db.prisma.clientPreviewFeatures` field:
main.wasp
```
app MyApp { // ... db: { system: PostgreSQL, prisma: { clientPreviewFeatures: ["postgresqlExtensions"] } }}
```
Read more about Prisma preview features in the [Prisma docs](https://www.prisma.io/docs/concepts/components/preview-features/client-preview-features).
### PostgreSQL Extensions[](#postgresql-extensions "Direct link to PostgreSQL Extensions")
PostgreSQL supports [extensions](https://www.postgresql.org/docs/current/contrib.html) that add additional functionality to the database. For example, the [hstore](https://www.postgresql.org/docs/13/hstore.html) extension adds support for storing key-value pairs in a single column.
To use PostgreSQL extensions with Prisma, you have to enable them in the `app.db.prisma.dbExtensions` field:
main.wasp
```
app MyApp { // ... db: { system: PostgreSQL, prisma: { clientPreviewFeatures: ["postgresqlExtensions"] dbExtensions: [ { name: "hstore", schema: "myHstoreSchema" }, { name: "pg_trgm" }, { name: "postgis", version: "2.1" }, ] } }}
```
Read more about PostgreSQL configuration in Wasp in the [API Reference](#the-appdb-field).
## API Reference[](#api-reference "Direct link to API Reference")
You can tell Wasp which database to use in the `app` declaration's `db` field:
### The `app.db` Field[](#the-appdb-field "Direct link to the-appdb-field")
Here's an example that uses the `app.db` field to its full potential:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { title: "My app", // ... db: { system: PostgreSQL, seeds: [ import devSeed from "@server/dbSeeds.js" ], prisma: { clientPreviewFeatures: ["extendedWhereUnique"] } }}
```
`app.db` is a dictionary with the following fields (all fields are optional):
* `system: DbSystem`
The database system Wasp should use. It can be either PostgreSQL or SQLite. The default value for the field is SQLite (this default value also applies if the entire `db` field is left unset). Whenever you modify the `db.system` field, make sure to run `wasp db migrate-dev` to apply the changes.
* `seeds: [ServerImport]`
Defines the seed functions you can use with the `wasp db seed` command to seed your database with initial data. Read the [Seeding section](#seeding-the-database) for more details.
* `prisma: PrismaOptions`
Additional configuration for Prisma.
main.wasp
```
app MyApp { // ... db: { // ... prisma: { clientPreviewFeatures: ["postgresqlExtensions"], dbExtensions: [ { name: "hstore", schema: "myHstoreSchema" }, { name: "pg_trgm" }, { name: "postgis", version: "2.1" }, ] } }}
```
It's a dictionary with the following fields:
* `clientPreviewFeatures : [string]`
Allows you to define [Prisma client preview features](https://www.prisma.io/docs/concepts/components/preview-features/client-preview-features), like for example, `"postgresqlExtensions"`.
* `dbExtensions: DbExtension[]`
It allows you to define PostgreSQL extensions that should be enabled for your database. Read more about [PostgreSQL extensions in Prisma](https://www.prisma.io/docs/concepts/components/prisma-schema/postgresql-extensions).
For each extension you define a dict with the following fields:
* `name: string` required
The name of the extension you would normally put in the Prisma file.
schema.prisma
```
extensions = [hstore(schema: "myHstoreSchema"), pg_trgm, postgis(version: "2.1")]// 👆 Extension name
```
* `map: string`
It sets the `map` argument of the extension. Explanation for the field from the Prisma docs:
> This is the database name of the extension. If this argument is not specified, the name of the extension in the Prisma schema must match the database name.
* `schema: string`
It sets the `schema` argument of the extension. Explanation for the field from the Prisma docs:
> This is the name of the schema in which to activate the extension's objects. If this argument is not specified, the current default object creation schema is used.
* `version: string`
It sets the `version` argument of the extension. Explanation for the field from the Prisma docs:
> This is the version of the extension to activate. If this argument is not specified, the value given in the extension's control file is used.
### CLI Commands for Seeding the Database[](#cli-commands-for-seeding-the-database "Direct link to CLI Commands for Seeding the Database")
Use one of the following commands to run the seed functions:
* `wasp db seed`
If you've only defined a single seed function, this command runs it. If you've defined multiple seed functions, it asks you to choose one interactively.
* `wasp db seed <seed-name>`
This command runs the seed function with the specified name. The name is the identifier used in its `import` expression in the `app.db.seeds` list. For example, to run the seed function `devSeedSimple` which was defined like this:
* JavaScript
* TypeScript
main.wasp
```
app MyApp { // ... db: { // ... seeds: [ // ... import { devSeedSimple } from "@server/dbSeeds.js", ] }}
```
Use the following command:
```
wasp db seed devSeedSimple
``` | null | https://wasp-lang.dev/docs/0.11.8/data-model/backends | Entities, Operations and Automatic CRUD together make a high-level interface for working with your app's data. Still, all that data has to live somewhere, so let's see how Wasp deals with databases. | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecfec89b20809-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:16:00 GMT | Tue, 23 Apr 2024 15:26:00 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=8YNz%2F%2BYvNBx7hDgCLlmNVEQzT5jB6LcfCywCk%2F9kCsIO3%2BlSxvLfnF2vpLSyJzG2kGDUvzas6KSEVLDvHGvhfBLbEsRLNZb9G4LET6WszwyXOGKULzM8rVGtZwSFmS3V"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 1cac898d7e52a925154e797173046dac986ffbca | null | 6DB8:2405A0:3CD26F8:47B3548:6627D0AF | null | MISS | cache-iad-kiad7000144-IAD | S1713885360.116988,VS0,VE14 | null | null | en | og:image | https://wasp-lang.dev/docs/0.11.8/data-model/backends | og:url | Databases | Wasp | og:title | Entities, Operations and Automatic CRUD together make a high-level interface for working with your app's data. Still, all that data has to live somewhere, so let's see how Wasp deals with databases. | og:description | Databases | Wasp | null | Databases
Entities, Operations and Automatic CRUD together make a high-level interface for working with your app's data. Still, all that data has to live somewhere, so let's see how Wasp deals with databases.
Supported Database Backends
Wasp supports multiple database backends. We'll list and explain each one.
SQLite
The default database Wasp uses is SQLite.
SQLite is a great way for getting started with a new project because it doesn't require any configuration, but Wasp can only use it in development. Once you want to deploy your Wasp app to production, you'll need to switch to PostgreSQL and stick with it.
Fortunately, migrating from SQLite to PostgreSQL is pretty simple, and we have a guide to help you.
PostgreSQL
PostgreSQL is the most advanced open source database and the fourth most popular database overall. It's been in active development for 20 years. Therefore, if you're looking for a battle-tested database, look no further.
To use Wasp with PostgreSQL, you'll have to ensure a database instance is running during development. Wasp needs access to your database for commands such as wasp start or wasp db migrate-dev and expects to find a connection string in the DATABASE_URL environment variable.
We cover all supported ways of connecting to a database in the next section.
Migrating from SQLite to PostgreSQL
To run your Wasp app in production, you'll need to switch from SQLite to PostgreSQL.
Set the app.db.system field to PostgreSQL.
main.wasp
app MyApp {
title: "My app",
// ...
db: {
system: PostgreSQL,
// ...
}
}
Delete all the old migrations, since they are SQLite migrations and can't be used with PostgreSQL, as well as the SQLite database by running wasp clean:
rm -r migrations/
wasp clean
Ensure your new database is running (check the section on connecing to a database to see how). Leave it running, since we need it for the next step.
In a different terminal, run wasp db migrate-dev to apply the changes and create a new initial migration.
That is it, you are all done!
Connecting to a Database
Assuming you're not using SQLite, Wasp offers two ways of connecting your app to a database instance:
A ready-made dev database that requires minimal setup and is great for quick prototyping.
A "real" database Wasp can connect to and use in production.
Using the Dev Database Provided by Wasp
The command wasp start db will start a default PostgreSQL dev database for you.
Your Wasp app will automatically connect to it, just keep wasp start db running in the background. Also, make sure that:
You have Docker installed and in PATH.
The port 5432 isn't taken.
Connecting to an existing database
If you want to spin up your own dev database (or connect to an external one), you can tell Wasp about it using the DATABASE_URL environment variable. Wasp will use the value of DATABASE_URL as a connection string.
The easiest way to set the necessary DATABASE_URL environment variable is by adding it to the .env.server file in the root dir of your Wasp project (if that file doesn't yet exist, create it).
Alternatively, you can set it inline when running wasp (this applies to all environment variables):
DATABASE_URL=<my-db-url> wasp ...
This trick is useful for running a certain wasp command on a specific database. For example, you could do:
DATABASE_URL=<production-db-url> wasp db seed myProductionSeed
This command seeds the data for a fresh staging or production database. To more precisely understand how seeding works, keep reading.
Seeding the Database
Database seeding is a term used for populating the database with some initial data.
Seeding is most commonly used for two following scenarios:
To put the development database into a state convenient for working and testing.
To initialize any database (dev, staging, or prod) with essential data it requires to operate. For example, populating the Currency table with default currencies, or the Country table with all available countries.
Writing a Seed Function
You can define as many seed functions as you want in an array under the app.db.seeds field:
JavaScript
TypeScript
main.wasp
app MyApp {
// ...
db: {
// ...
seeds: [
import { devSeedSimple } from "@server/dbSeeds.js",
import { prodSeed } from "@server/dbSeeds.js"
]
}
}
Each seed function must be an async function that takes one argument, prismaClient, which is a Prisma Client instance used to interact with the database. This is the same Prisma Client instance that Wasp uses internally and thus includes all of the usual features (e.g., password hashing).
Since a seed function falls under server-side code, it can import other server-side functions. This is convenient because you might want to seed the database using Actions.
Here's an example of a seed function that imports an Action:
JavaScript
TypeScript
import { createTask } from "./actions.js";
export const devSeedSimple = async (prismaClient) => {
const user = await createUser(prismaClient, {
username: "RiuTheDog",
password: "bark1234",
});
await createTask(
{ description: "Chase the cat" },
{ user, entities: { Task: prismaClient.task } }
);
};
async function createUser(prismaClient, data) {
const { password, ...newUser } = await prismaClient.user.create({ data });
return newUser;
}
Running seed functions
Run the command wasp db seed and Wasp will ask you which seed function you'd like to run (if you've defined more than one).
Alternatively, run the command wasp db seed <seed-name> to choose a specific seed function right away, for example:
wasp db seed devSeedSimple
Check the API Reference for more details on these commands.
tip
You'll often want to call wasp db seed right after you run wasp db reset, as it makes sense to fill the database with initial data after clearing it.
Prisma Configuration
Wasp uses Prisma to interact with the database. Prisma is a "Next-generation Node.js and TypeScript ORM" that provides a type-safe API for working with your database.
Prisma Preview Features
Prisma is still in active development and some of its features are not yet stable. To use them, you have to enable them in the app.db.prisma.clientPreviewFeatures field:
main.wasp
app MyApp {
// ...
db: {
system: PostgreSQL,
prisma: {
clientPreviewFeatures: ["postgresqlExtensions"]
}
}
}
Read more about Prisma preview features in the Prisma docs.
PostgreSQL Extensions
PostgreSQL supports extensions that add additional functionality to the database. For example, the hstore extension adds support for storing key-value pairs in a single column.
To use PostgreSQL extensions with Prisma, you have to enable them in the app.db.prisma.dbExtensions field:
main.wasp
app MyApp {
// ...
db: {
system: PostgreSQL,
prisma: {
clientPreviewFeatures: ["postgresqlExtensions"]
dbExtensions: [
{ name: "hstore", schema: "myHstoreSchema" },
{ name: "pg_trgm" },
{ name: "postgis", version: "2.1" },
]
}
}
}
Read more about PostgreSQL configuration in Wasp in the API Reference.
API Reference
You can tell Wasp which database to use in the app declaration's db field:
The app.db Field
Here's an example that uses the app.db field to its full potential:
JavaScript
TypeScript
main.wasp
app MyApp {
title: "My app",
// ...
db: {
system: PostgreSQL,
seeds: [
import devSeed from "@server/dbSeeds.js"
],
prisma: {
clientPreviewFeatures: ["extendedWhereUnique"]
}
}
}
app.db is a dictionary with the following fields (all fields are optional):
system: DbSystem
The database system Wasp should use. It can be either PostgreSQL or SQLite. The default value for the field is SQLite (this default value also applies if the entire db field is left unset). Whenever you modify the db.system field, make sure to run wasp db migrate-dev to apply the changes.
seeds: [ServerImport]
Defines the seed functions you can use with the wasp db seed command to seed your database with initial data. Read the Seeding section for more details.
prisma: PrismaOptions
Additional configuration for Prisma.
main.wasp
app MyApp {
// ...
db: {
// ...
prisma: {
clientPreviewFeatures: ["postgresqlExtensions"],
dbExtensions: [
{ name: "hstore", schema: "myHstoreSchema" },
{ name: "pg_trgm" },
{ name: "postgis", version: "2.1" },
]
}
}
}
It's a dictionary with the following fields:
clientPreviewFeatures : [string]
Allows you to define Prisma client preview features, like for example, "postgresqlExtensions".
dbExtensions: DbExtension[]
It allows you to define PostgreSQL extensions that should be enabled for your database. Read more about PostgreSQL extensions in Prisma.
For each extension you define a dict with the following fields:
name: string required
The name of the extension you would normally put in the Prisma file.
schema.prisma
extensions = [hstore(schema: "myHstoreSchema"), pg_trgm, postgis(version: "2.1")]
// 👆 Extension name
map: string
It sets the map argument of the extension. Explanation for the field from the Prisma docs:
This is the database name of the extension. If this argument is not specified, the name of the extension in the Prisma schema must match the database name.
schema: string
It sets the schema argument of the extension. Explanation for the field from the Prisma docs:
This is the name of the schema in which to activate the extension's objects. If this argument is not specified, the current default object creation schema is used.
version: string
It sets the version argument of the extension. Explanation for the field from the Prisma docs:
This is the version of the extension to activate. If this argument is not specified, the value given in the extension's control file is used.
CLI Commands for Seeding the Database
Use one of the following commands to run the seed functions:
wasp db seed
If you've only defined a single seed function, this command runs it. If you've defined multiple seed functions, it asks you to choose one interactively.
wasp db seed <seed-name>
This command runs the seed function with the specified name. The name is the identifier used in its import expression in the app.db.seeds list. For example, to run the seed function devSeedSimple which was defined like this:
JavaScript
TypeScript
main.wasp
app MyApp {
// ...
db: {
// ...
seeds: [
// ...
import { devSeedSimple } from "@server/dbSeeds.js",
]
}
}
Use the following command:
wasp db seed devSeedSimple | https://wasp-lang.dev/docs/0.11.8/data-model/backends |
|
2 | null | 2024-04-23T15:16:02.419Z | https://wasp-lang.dev/docs/0.12.0/quick-start | https://wasp-lang.dev/docs/0.12.0 | http | ## Quick Start
## Installation[](#installation "Direct link to Installation")
Welcome, new Waspeteer 🐝!
Let's create and run our first Wasp app in 3 short steps:
1. **To install Wasp on Linux / OSX / WSL (Windows), open your terminal and run:**
```
curl -sSL https://get.wasp-lang.dev/installer.sh | sh
```
ℹ️ Wasp requires Node.js and will warn you if it is missing: check below for [more details](#requirements).
2. **Then, create a new app by running:**
3. **Finally, run the app:**
```
cd <my-project-name>wasp start
```
That's it 🎉 You have successfully created and served a new full-stack web app at [http://localhost:3000](http://localhost:3000/) and Wasp is serving both frontend and backend for you.
Something Unclear?
Check [More Details](#more-details) section below if anything went wrong with the installation, or if you have additional questions.
Want an even faster start?
Try out [Wasp AI](https://wasp-lang.dev/docs/0.12.0/wasp-ai/creating-new-app) 🤖 to generate a new Wasp app in minutes just from a title and short description!
Try Wasp Without Installing 🤔?
Give Wasp a spin in the browser without any setup by running our [Wasp Template for Gitpod](https://github.com/wasp-lang/gitpod-template)
### What next?[](#what-next "Direct link to What next?")
* 👉 **Check out the [Todo App tutorial](https://wasp-lang.dev/docs/0.12.0/tutorial/create), which will take you through all the core features of Wasp!** 👈
* [Setup your editor](https://wasp-lang.dev/docs/0.12.0/editor-setup) for working with Wasp.
* Join us on [Discord](https://discord.gg/rzdnErX)! Any feedback or questions you have, we are there for you.
* Follow Wasp development by subscribing to our newsletter: [https://wasp-lang.dev/#signup](https://wasp-lang.dev/#signup) . We usually send 1 per month, and [Matija](https://github.com/matijaSos) does his best to unleash his creativity to make them engaging and fun to read :D!
* * *
## More details[](#more-details "Direct link to More details")
### Requirements[](#requirements "Direct link to Requirements")
You must have Node.js (and NPM) installed on your machine and available in `PATH`. A version of Node.js must be >= 18.
If you need it, we recommend using [nvm](https://github.com/nvm-sh/nvm) for managing your Node.js installation version(s).
A quick guide on installing/using nvm
Install nvm via your OS package manager (`apt`, `pacman`, `homebrew`, ...) or via the [nvm](https://github.com/nvm-sh/nvm#install--update-script) install script.
Then, install a version of Node.js that you need:
Finally, whenever you need to ensure a specific version of Node.js is used, run:
to set the Node.js version for the current shell session.
You can run
to check the version of Node.js currently being used in this shell session.
Check NVM repo for more details: [https://github.com/nvm-sh/nvm](https://github.com/nvm-sh/nvm).
### Installation[](#installation-1 "Direct link to Installation")
* Linux / macOS
* Windows
* From source
Open your terminal and run:
```
curl -sSL https://get.wasp-lang.dev/installer.sh | sh
```
Running Wasp on Mac with Mx chip (arm64)
**Experiencing the 'Bad CPU type in executable' issue on a device with arm64 (Apple Silicon)?** Given that the wasp binary is built for x86 and not for arm64 (Apple Silicon), you'll need to install [Rosetta on your Mac](https://support.apple.com/en-us/HT211861) if you are using a Mac with Mx (M1, M2, ...). Rosetta is a translation process that enables users to run applications designed for x86 on arm64 (Apple Silicon). To install Rosetta, run the following command in your terminal
```
softwareupdate --install-rosetta
```
Once Rosetta is installed, you should be able to run Wasp without any issues. | null | https://wasp-lang.dev/docs/0.12.0/quick-start | Installation | * | 0 | h3=":443"; ma=86400 | max-age=600 | DYNAMIC | 878ecffacb99389e-IAD | close | br | text/html; charset=utf-8 | Tue, 23 Apr 2024 15:16:02 GMT | Tue, 23 Apr 2024 15:26:02 GMT | Thu, 18 Apr 2024 15:50:26 GMT | {"success_fraction":0,"report_to":"cf-nel","max_age":604800} | {"endpoints":[{"url":"https:\/\/a.nel.cloudflare.com\/report\/v4?s=V%2BQUR%2BJes2AfCb9KubAbbg49bqF407rnNXAHYYMxkEIay0xQHf6d2OtpUlQII%2FzMFoawG2cVnbbhwXb8QiM5%2F0FKRMZWZk5vCGZlw4uqi8lMQk5YFDkpLjhCIHOWovYa"}],"group":"cf-nel","max_age":604800} | cloudflare | chunked | Accept-Encoding | 1.1 varnish | MISS | 0 | 5026d66c688deba8d49017dbce1054a2e81b8ae8 | null | 44EE:EB4F4:3BEBE47:46F2377:6627D0B2 | HIT | MISS | cache-iad-kiad7000132-IAD | S1713885362.379405,VS0,VE11 | null | null | en | og:image | https://wasp-lang.dev/docs/0.12.0/quick-start | og:url | Quick Start | Wasp | og:title | Installation | og:description | Quick Start | Wasp | null | Quick Start
Installation
Welcome, new Waspeteer 🐝!
Let's create and run our first Wasp app in 3 short steps:
To install Wasp on Linux / OSX / WSL (Windows), open your terminal and run:
curl -sSL https://get.wasp-lang.dev/installer.sh | sh
ℹ️ Wasp requires Node.js and will warn you if it is missing: check below for more details.
Then, create a new app by running:
Finally, run the app:
cd <my-project-name>
wasp start
That's it 🎉 You have successfully created and served a new full-stack web app at http://localhost:3000 and Wasp is serving both frontend and backend for you.
Something Unclear?
Check More Details section below if anything went wrong with the installation, or if you have additional questions.
Want an even faster start?
Try out Wasp AI 🤖 to generate a new Wasp app in minutes just from a title and short description!
Try Wasp Without Installing 🤔?
Give Wasp a spin in the browser without any setup by running our Wasp Template for Gitpod
What next?
👉 Check out the Todo App tutorial, which will take you through all the core features of Wasp! 👈
Setup your editor for working with Wasp.
Join us on Discord! Any feedback or questions you have, we are there for you.
Follow Wasp development by subscribing to our newsletter: https://wasp-lang.dev/#signup . We usually send 1 per month, and Matija does his best to unleash his creativity to make them engaging and fun to read :D!
More details
Requirements
You must have Node.js (and NPM) installed on your machine and available in PATH. A version of Node.js must be >= 18.
If you need it, we recommend using nvm for managing your Node.js installation version(s).
A quick guide on installing/using nvm
Install nvm via your OS package manager (apt, pacman, homebrew, ...) or via the nvm install script.
Then, install a version of Node.js that you need:
Finally, whenever you need to ensure a specific version of Node.js is used, run:
to set the Node.js version for the current shell session.
You can run
to check the version of Node.js currently being used in this shell session.
Check NVM repo for more details: https://github.com/nvm-sh/nvm.
Installation
Linux / macOS
Windows
From source
Open your terminal and run:
curl -sSL https://get.wasp-lang.dev/installer.sh | sh
Running Wasp on Mac with Mx chip (arm64)
Experiencing the 'Bad CPU type in executable' issue on a device with arm64 (Apple Silicon)? Given that the wasp binary is built for x86 and not for arm64 (Apple Silicon), you'll need to install Rosetta on your Mac if you are using a Mac with Mx (M1, M2, ...). Rosetta is a translation process that enables users to run applications designed for x86 on arm64 (Apple Silicon). To install Rosetta, run the following command in your terminal
softwareupdate --install-rosetta
Once Rosetta is installed, you should be able to run Wasp without any issues. | https://wasp-lang.dev/docs/0.12.0/quick-start |