text
stringlengths 2
99k
| meta
dict |
---|---|
/*
* Copyright (c) 2002-2020 "Neo4j,"
* Neo4j Sweden AB [http://neo4j.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
package org.neo4j.kernel.api.impl.fulltext.analyzer.providers;
import org.apache.lucene.analysis.Analyzer;
import org.apache.lucene.analysis.es.SpanishAnalyzer;
import org.neo4j.annotations.service.ServiceProvider;
import org.neo4j.graphdb.schema.AnalyzerProvider;
@ServiceProvider
public class Spanish extends AnalyzerProvider
{
public Spanish()
{
super( "spanish" );
}
@Override
public Analyzer createAnalyzer()
{
return new SpanishAnalyzer();
}
@Override
public String description()
{
return "Spanish analyzer with stemming and stop word filtering.";
}
}
| {
"pile_set_name": "Github"
} |
---
-api-id: M:Windows.Storage.IStorageFolder.CreateFileAsync(System.String)
-api-type: winrt method
---
<!-- Method syntax
public Windows.Foundation.IAsyncOperation<Windows.Storage.StorageFile> CreateFileAsync(System.String desiredName)
-->
# Windows.Storage.IStorageFolder.CreateFileAsync
## -description
Creates a new file in the current folder.
## -parameters
### -param desiredName
The desired name of the file to create.
## -returns
When this method completes, it returns the new file as a [StorageFile](storagefile.md).
## -remarks
## -examples
## -see-also
[CreateFileAsync(String, CreationCollisionOption)](istoragefolder_createfileasync_1058061470.md), [StorageFolder.CreateFileAsync](/uwp/api/windows.storage.storagefolder.createfileasync) | {
"pile_set_name": "Github"
} |
# 题目描述(中等难度)
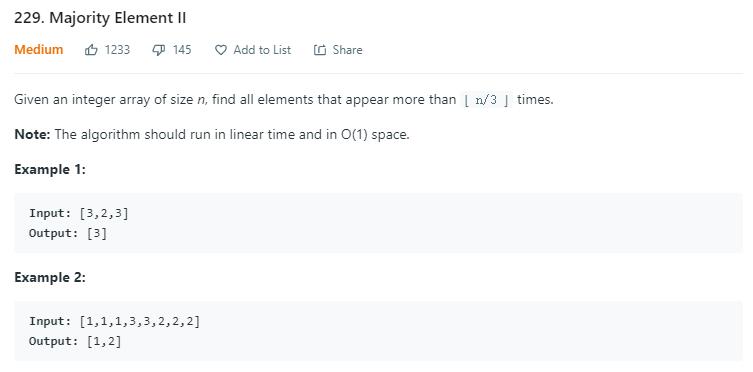
找出数组中数量超过 `n/3` 的数字,`n` 是数组的长度。
# 解法一
题目要求是 `O(1)` 的空间复杂度,我们先用 `map` 写一下,看看对题意的理解对不对。
`map` 的话 `key` 存数字,`value` 存数字出现的个数。如果数字出现的次数等于了 `n/3 + 1` 就把它加到结果中。
```java
public List<Integer> majorityElement(int[] nums) {
int n = nums.length;
HashMap<Integer, Integer> map = new HashMap<>();
List<Integer> res = new ArrayList<>();
for (int i = 0; i < n; i++) {
int count = map.getOrDefault(nums[i], 0);
//之前的数量已经是 n/3, 当前数量就是 n/3 + 1 了
if (count == n / 3) {
res.add(nums[i]);
}
//可以提前结束
if (count == 2 * n / 3 || res.size() == 2) {
return res;
}
map.put(nums[i], count + 1);
}
return res;
}
```
# 解法二
[169 题](https://leetcode.wang/leetcode-169-Majority-Element.html) 我们做过找出数组的中超过 `n/2` 数量的数字,其中介绍了摩尔投票法,这里的话可以改写一下,参考 [这里](https://leetcode.com/problems/majority-element-ii/discuss/63520/Boyer-Moore-Majority-Vote-algorithm-and-my-elaboration)。
首先看一下 [169 题](https://leetcode.wang/leetcode-169-Majority-Element.html) 我们是怎么做的。
> 我们假设这样一个场景,在一个游戏中,分了若干个队伍,有一个队伍的人数超过了半数。所有人的战力都相同,不同队伍的两个人遇到就是同归于尽,同一个队伍的人遇到当然互不伤害。
>
> 这样经过充分时间的游戏后,最后的结果是确定的,一定是超过半数的那个队伍留在了最后。
>
> 而对于这道题,我们只需要利用上边的思想,把数组的每个数都看做队伍编号,然后模拟游戏过程即可。
>
> `group` 记录当前队伍的人数,`count` 记录当前队伍剩余的人数。如果当前队伍剩余人数为 `0`,记录下次遇到的人的所在队伍号。
对于这道题的话,超过 `n/3` 的队伍可能有两个,首先我们用 `group1` 和 `group2` 记录这两个队伍,`count1` 和 `count2` 分别记录两个队伍的数量,然后遵循下边的游戏规则。
将数组中的每一个数字看成队伍编号。
`group1` 和 `group2` 首先初始化为不可能和当前数字相等的两个数,将这两个队伍看成同盟,它俩不互相伤害。
然后遍历数组中的其他数字,如果遇到的数字属于其中的一个队伍,就将当前队伍的数量加 `1`。
如果某个队伍的数量变成了 `0`,就把这个队伍编号更新为当前的数字。
否则的话,将两个队伍的数量都减 `1`。
```java
public List<Integer> majorityElement(int[] nums) {
int n = nums.length;
long group1 = (long)Integer.MAX_VALUE + 1;
int count1 = 0;
long group2 = (long)Integer.MAX_VALUE + 1;
int count2 = 0;
for (int i = 0; i < n; i++) {
if (nums[i] == group1) {
count1++;
} else if (nums[i] == group2) {
count2++;
} else if (count1 == 0) {
group1 = nums[i];
count1 = 1;
} else if (count2 == 0) {
group2 = nums[i];
count2 = 1;
} else {
count1--;
count2--;
}
}
//计算两个队伍的数量,因为可能只存在一个数字的数量超过了 n/3
count1 = 0;
count2 = 0;
for (int i = 0; i < n; i++) {
if (nums[i] == group1) {
count1++;
}
if (nums[i] == group2) {
count2++;
}
}
//只保存数量大于 n/3 的队伍
List<Integer> res = new ArrayList<>();
if (count1 > n / 3) {
res.add((int) group1);
}
if (count2 > n / 3) {
res.add((int) group2);
}
return res;
}
```
上边有个技巧就是先将 `group` 初始化为一个大于 `int` 最大值的 `long` 值,这样可以保证后边的 `if` 条件判断中,数组中一定不会有数字和 `group`相等,从而进入后边的更新队伍编号的分支中。除了用 `long` 值,我们还可以用包装对象 `Integer`,将 `group` 初始化为 `null` 可以达到同样的效果。
当然,不用上边的技巧也是可以的,我们可以先在 `nums` 里找到两个不同的值分别赋值给 `group1` 和 `group2` 中即可,只不过代码上不会有上边的简洁。
`2020.5.27` 更新,[@Frankie](http://yaoyichen.cn/algorithm/2020/05/27/leetcode-229.html) 提醒,其实不用上边分析的那么麻烦,只需要给 `group1` 和 `group2` 随便赋两个不相等的值即可。
因为如果数组中前两个数有和 `group1` 或者 `group2` 相等的元素,就进入前两个 `if` 语句中的某一个,逻辑上也没问题。
如果数组中前两个数没有和 `group1` 或者 `group2` 相等的元素,那么就和使用 `long` 一个性质了。
```java
public List<Integer> majorityElement(int[] nums) {
int n = nums.length;
int group1 = 0;
int count1 = 0;
int group2 = 1;
int count2 = 0;
for (int i = 0; i < n; i++) {
if (nums[i] == group1) {
count1++;
} else if (nums[i] == group2) {
count2++;
} else if (count1 == 0) {
group1 = nums[i];
count1 = 1;
} else if (count2 == 0) {
group2 = nums[i];
count2 = 1;
} else {
count1--;
count2--;
}
}
//计算两个队伍的数量,因为可能只存在一个数字的数量超过了 n/3
count1 = 0;
count2 = 0;
for (int i = 0; i < n; i++) {
if (nums[i] == group1) {
count1++;
}
if (nums[i] == group2) {
count2++;
}
}
//只保存数量大于 n/3 的队伍
List<Integer> res = new ArrayList<>();
if (count1 > n / 3) {
res.add( group1);
}
if (count2 > n / 3) {
res.add(group2);
}
return res;
}
```
# 总
解法一算是通用的解法,解法二的话看起来比较容易,但如果只看上边的解析,然后自己写代码的话还是会遇到很多问题的,其中 `if` 分支的顺序很重要。 | {
"pile_set_name": "Github"
} |
#!/bin/bash
exitflg=0
if type fontforge > /dev/null 2>&1; then
echo '[DSEG]Fontforge is detected.'
else
echo '[DSEG]Please install fontforge.(https://fontforge.github.io/en-US/)'
exitflg=1
fi
if type woff2_compress > /dev/null 2>&1; then
echo '[DSEG]Google woff2 is detected.'
else
echo '[DSEG]Please install google woff2.(https://github.com/google/woff2)'
exitflg=1
fi
if [ $exitflg -eq 1 ]; then
exit 1
else
for a in src/*.sfd; do
fontfile=$(basename $a .sfd)
namedir=$(echo $fontfile | sed "s,-.*,," | sed "s,Mini,-MINI,g;s,Modern,-Modern,;s,Classic,-Classic,;s,7SEGG,-7SEGG-,")
name=$(echo $fontfile | sed "s,-.*,,")
type=$(echo $fontfile | sed "s,.*-,,")
mkdir -p fonts/${namedir}/
fontforge -lang=ff -c 'Open($1); Generate($2)' src/${fontfile}.sfd fonts/$namedir/${name}-${type}.ttf >&/dev/null
fontforge -lang=ff -c 'Open($1); Generate($2)' src/${fontfile}.sfd fonts/$namedir/${name}-${type}.woff >&/dev/null
woff2_compress fonts/$namedir/${name}-${type}.ttf >&/dev/null
done
for b in fonts/DSEGWeather/DSEGWeather-DSEGWeather*; do
ext=$(echo $b | sed 's/^.*\.\([^\.]*\)$/\1/')
mv fonts/DSEGWeather/DSEGWeather-DSEGWeather.${ext} fonts/DSEGWeather/DSEGWeather.${ext}
done
mv fonts/DSEG7-7SEGG-CHANMINI/* fonts/DSEG7-7SEGG-CHAN
rm -r fonts/DSEG7-7SEGG-CHANMINI
for c in fonts/DSEG7-7SEGG-CHAN/DSEG77S*; do
file=$(basename $c)
fn=$(echo $file | sed 's/DSEG77S/DSEG7S/')
mv fonts/DSEG7-7SEGG-CHAN/${file} fonts/DSEG7-7SEGG-CHAN/${fn}
done
echo '[DSEG]DSEG font file is generated.'
fi
| {
"pile_set_name": "Github"
} |
#pragma once
#include "MarmaladePlugIn.h"
class CMarmaladeRMP;
class CMarmaladeRdkPlugIn : public CRhRdkRenderPlugIn
{
public:
CMarmaladeRdkPlugIn(void);
virtual ~CMarmaladeRdkPlugIn(void);
// Initialization.
virtual bool Initialize(void) override;
virtual void Uninitialize(void) override;
virtual UUID PlugInId(void) const override;
CRhinoPlugIn& RhinoPlugIn(void) const override { return ::MarmaladePlugIn(); }
protected:
virtual bool AllowChooseContent(const CRhRdkContent& content) const override;
virtual void RegisterExtensions(void) const override;
virtual void AbortRender(void) override;
virtual CRhRdkVariant GetParameter(const wchar_t* wszParam) const override;
// Preview renderers.
virtual bool CreatePreview(const ON_2iSize& sizeImage, RhRdkPreviewQuality quality,
const IRhRdkPreviewSceneServer* pSceneServer, IRhRdkPreviewCallbacks* pNotify, CRhinoDib& dibOut) override;
virtual bool CreatePreview(const ON_2iSize& sizeImage, const CRhRdkTexture& texture, CRhinoDib& dibOut) override;
virtual bool SupportsFeature(const UUID& uuidFeature) const override;
virtual void AddCustomRenderSettingsSections(RhRdkUiModalities m, ON_SimpleArray<IRhinoUiSection*>& aSections) const override;
#ifdef OBSOLETE
void PlugInRdkVersion(int& iMajor, int& iMinor, int& iBeta) const { iMajor = RDK_MAJOR_VERSION; iMinor = RDK_MINOR_VERSION, iBeta = RDK_BETA_RELEASE; }
virtual void AddCustomEditorActions(IRhRdkActions& actions, const IRhRdkContentEditor& editor) const override;
virtual void UpdateCustomEditorActions(IRhRdkActions& actions, const IRhRdkContentEditor& editor) const override;
virtual void AddCustomEditorMenu(IRhRdkMenu& menu, const IRhRdkContentEditor& editor) const override;
#endif
};
| {
"pile_set_name": "Github"
} |
// SPDX-License-Identifier: GPL-2.0
#include <linux/compiler.h>
static int arc__annotate_init(struct arch *arch, char *cpuid __maybe_unused)
{
arch->initialized = true;
arch->objdump.comment_char = ';';
return 0;
}
| {
"pile_set_name": "Github"
} |
/** \file
* \ingroup smoke
*/
//////////////////////////////////////////////////////////////////////
// This file is part of Wavelet Turbulence.
//
// Wavelet Turbulence is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Wavelet Turbulence is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with Wavelet Turbulence. If not, see <http://www.gnu.org/licenses/>.
//
// Copyright 2008 Theodore Kim and Nils Thuerey
//
// FLUID_3D.cpp: implementation of the FLUID_3D class.
//
//////////////////////////////////////////////////////////////////////
// Heavy parallel optimization done. Many of the old functions now
// take begin and end parameters and process only specified part of the data.
// Some functions were divided into multiple ones.
// - MiikaH
//////////////////////////////////////////////////////////////////////
#include "FLUID_3D.h"
#include "IMAGE.h"
#include <INTERPOLATE.h>
#include "SPHERE.h"
#include <zlib.h>
#include "float.h"
#if PARALLEL==1
#include <omp.h>
#endif // PARALLEL
//////////////////////////////////////////////////////////////////////
// Construction/Destruction
//////////////////////////////////////////////////////////////////////
FLUID_3D::FLUID_3D(int *res, float dx, float dtdef, int init_heat, int init_fire, int init_colors) :
_xRes(res[0]), _yRes(res[1]), _zRes(res[2]), _res(0.0f)
{
// set simulation consts
_dt = dtdef; // just in case. set in step from a RNA factor
_iterations = 100;
_tempAmb = 0;
_heatDiffusion = 1e-3;
_totalTime = 0.0f;
_totalSteps = 0;
_res = Vec3Int(_xRes,_yRes,_zRes);
_maxRes = MAX3(_xRes, _yRes, _zRes);
// initialize wavelet turbulence
/*
if(amplify)
_wTurbulence = new WTURBULENCE(_res[0],_res[1],_res[2], amplify, noisetype);
else
_wTurbulence = NULL;
*/
// scale the constants according to the refinement of the grid
if (!dx)
_dx = 1.0f / (float)_maxRes;
else
_dx = dx;
_constantScaling = 64.0f / _maxRes;
_constantScaling = (_constantScaling < 1.0f) ? 1.0f : _constantScaling;
_vorticityEps = 2.0f / _constantScaling; // Just in case set a default value
// allocate arrays
_totalCells = _xRes * _yRes * _zRes;
_slabSize = _xRes * _yRes;
_xVelocity = new float[_totalCells];
_yVelocity = new float[_totalCells];
_zVelocity = new float[_totalCells];
_xVelocityOb = new float[_totalCells];
_yVelocityOb = new float[_totalCells];
_zVelocityOb = new float[_totalCells];
_xVelocityOld = new float[_totalCells];
_yVelocityOld = new float[_totalCells];
_zVelocityOld = new float[_totalCells];
_xForce = new float[_totalCells];
_yForce = new float[_totalCells];
_zForce = new float[_totalCells];
_density = new float[_totalCells];
_densityOld = new float[_totalCells];
_obstacles = new unsigned char[_totalCells]; // set 0 at end of step
// For threaded version:
_xVelocityTemp = new float[_totalCells];
_yVelocityTemp = new float[_totalCells];
_zVelocityTemp = new float[_totalCells];
_densityTemp = new float[_totalCells];
// DG TODO: check if alloc went fine
for (int x = 0; x < _totalCells; x++)
{
_density[x] = 0.0f;
_densityOld[x] = 0.0f;
_xVelocity[x] = 0.0f;
_yVelocity[x] = 0.0f;
_zVelocity[x] = 0.0f;
_xVelocityOb[x] = 0.0f;
_yVelocityOb[x] = 0.0f;
_zVelocityOb[x] = 0.0f;
_xVelocityOld[x] = 0.0f;
_yVelocityOld[x] = 0.0f;
_zVelocityOld[x] = 0.0f;
_xForce[x] = 0.0f;
_yForce[x] = 0.0f;
_zForce[x] = 0.0f;
_obstacles[x] = false;
}
/* heat */
_heat = _heatOld = _heatTemp = NULL;
if (init_heat) {
initHeat();
}
// Fire simulation
_flame = _fuel = _fuelTemp = _fuelOld = NULL;
_react = _reactTemp = _reactOld = NULL;
if (init_fire) {
initFire();
}
// Smoke color
_color_r = _color_rOld = _color_rTemp = NULL;
_color_g = _color_gOld = _color_gTemp = NULL;
_color_b = _color_bOld = _color_bTemp = NULL;
if (init_colors) {
initColors(0.0f, 0.0f, 0.0f);
}
// boundary conditions of the fluid domain
// set default values -> vertically non-colliding
_domainBcFront = true;
_domainBcTop = false;
_domainBcLeft = true;
_domainBcBack = _domainBcFront;
_domainBcBottom = _domainBcTop;
_domainBcRight = _domainBcLeft;
_colloPrev = 1; // default value
}
void FLUID_3D::initHeat()
{
if (!_heat) {
_heat = new float[_totalCells];
_heatOld = new float[_totalCells];
_heatTemp = new float[_totalCells];
for (int x = 0; x < _totalCells; x++)
{
_heat[x] = 0.0f;
_heatOld[x] = 0.0f;
}
}
}
void FLUID_3D::initFire()
{
if (!_flame) {
_flame = new float[_totalCells];
_fuel = new float[_totalCells];
_fuelTemp = new float[_totalCells];
_fuelOld = new float[_totalCells];
_react = new float[_totalCells];
_reactTemp = new float[_totalCells];
_reactOld = new float[_totalCells];
for (int x = 0; x < _totalCells; x++)
{
_flame[x] = 0.0f;
_fuel[x] = 0.0f;
_fuelTemp[x] = 0.0f;
_fuelOld[x] = 0.0f;
_react[x] = 0.0f;
_reactTemp[x] = 0.0f;
_reactOld[x] = 0.0f;
}
}
}
void FLUID_3D::initColors(float init_r, float init_g, float init_b)
{
if (!_color_r) {
_color_r = new float[_totalCells];
_color_rOld = new float[_totalCells];
_color_rTemp = new float[_totalCells];
_color_g = new float[_totalCells];
_color_gOld = new float[_totalCells];
_color_gTemp = new float[_totalCells];
_color_b = new float[_totalCells];
_color_bOld = new float[_totalCells];
_color_bTemp = new float[_totalCells];
for (int x = 0; x < _totalCells; x++)
{
_color_r[x] = _density[x] * init_r;
_color_rOld[x] = 0.0f;
_color_g[x] = _density[x] * init_g;
_color_gOld[x] = 0.0f;
_color_b[x] = _density[x] * init_b;
_color_bOld[x] = 0.0f;
}
}
}
void FLUID_3D::setBorderObstacles()
{
// set side obstacles
unsigned int index;
for (int y = 0; y < _yRes; y++)
for (int x = 0; x < _xRes; x++)
{
// bottom slab
index = x + y * _xRes;
if(_domainBcBottom) _obstacles[index] = 1;
// top slab
index += _totalCells - _slabSize;
if(_domainBcTop) _obstacles[index] = 1;
}
for (int z = 0; z < _zRes; z++)
for (int x = 0; x < _xRes; x++)
{
// front slab
index = x + z * _slabSize;
if(_domainBcFront) _obstacles[index] = 1;
// back slab
index += _slabSize - _xRes;
if(_domainBcBack) _obstacles[index] = 1;
}
for (int z = 0; z < _zRes; z++)
for (int y = 0; y < _yRes; y++)
{
// left slab
index = y * _xRes + z * _slabSize;
if(_domainBcLeft) _obstacles[index] = 1;
// right slab
index += _xRes - 1;
if(_domainBcRight) _obstacles[index] = 1;
}
}
FLUID_3D::~FLUID_3D()
{
if (_xVelocity) delete[] _xVelocity;
if (_yVelocity) delete[] _yVelocity;
if (_zVelocity) delete[] _zVelocity;
if (_xVelocityOb) delete[] _xVelocityOb;
if (_yVelocityOb) delete[] _yVelocityOb;
if (_zVelocityOb) delete[] _zVelocityOb;
if (_xVelocityOld) delete[] _xVelocityOld;
if (_yVelocityOld) delete[] _yVelocityOld;
if (_zVelocityOld) delete[] _zVelocityOld;
if (_xForce) delete[] _xForce;
if (_yForce) delete[] _yForce;
if (_zForce) delete[] _zForce;
if (_density) delete[] _density;
if (_densityOld) delete[] _densityOld;
if (_heat) delete[] _heat;
if (_heatOld) delete[] _heatOld;
if (_obstacles) delete[] _obstacles;
if (_xVelocityTemp) delete[] _xVelocityTemp;
if (_yVelocityTemp) delete[] _yVelocityTemp;
if (_zVelocityTemp) delete[] _zVelocityTemp;
if (_densityTemp) delete[] _densityTemp;
if (_heatTemp) delete[] _heatTemp;
if (_flame) delete[] _flame;
if (_fuel) delete[] _fuel;
if (_fuelTemp) delete[] _fuelTemp;
if (_fuelOld) delete[] _fuelOld;
if (_react) delete[] _react;
if (_reactTemp) delete[] _reactTemp;
if (_reactOld) delete[] _reactOld;
if (_color_r) delete[] _color_r;
if (_color_rOld) delete[] _color_rOld;
if (_color_rTemp) delete[] _color_rTemp;
if (_color_g) delete[] _color_g;
if (_color_gOld) delete[] _color_gOld;
if (_color_gTemp) delete[] _color_gTemp;
if (_color_b) delete[] _color_b;
if (_color_bOld) delete[] _color_bOld;
if (_color_bTemp) delete[] _color_bTemp;
// printf("deleted fluid\n");
}
// init direct access functions from blender
void FLUID_3D::initBlenderRNA(float *alpha, float *beta, float *dt_factor, float *vorticity, int *borderCollision, float *burning_rate,
float *flame_smoke, float *flame_smoke_color, float *flame_vorticity, float *flame_ignition_temp, float *flame_max_temp)
{
_alpha = alpha;
_beta = beta;
_dtFactor = dt_factor;
_vorticityRNA = vorticity;
_borderColli = borderCollision;
_burning_rate = burning_rate;
_flame_smoke = flame_smoke;
_flame_smoke_color = flame_smoke_color;
_flame_vorticity = flame_vorticity;
_ignition_temp = flame_ignition_temp;
_max_temp = flame_max_temp;
}
//////////////////////////////////////////////////////////////////////
// step simulation once
//////////////////////////////////////////////////////////////////////
void FLUID_3D::step(float dt, float gravity[3])
{
#if 0
// If border rules have been changed
if (_colloPrev != *_borderColli) {
printf("Border collisions changed\n");
// DG TODO: Need to check that no animated obstacle flags are overwritten
setBorderCollisions();
}
#endif
// DG: TODO for the moment redo border for every timestep since it's been deleted every time by moving obstacles
setBorderCollisions();
// set delta time by dt_factor
_dt = (*_dtFactor) * dt;
// set vorticity from RNA value
_vorticityEps = (*_vorticityRNA)/_constantScaling;
#if PARALLEL==1
int threadval = 1;
threadval = omp_get_max_threads();
int stepParts = 1;
float partSize = _zRes;
stepParts = threadval*2; // Dividing parallelized sections into numOfThreads * 2 sections
partSize = (float)_zRes/stepParts; // Size of one part;
if (partSize < 4) {stepParts = threadval; // If the slice gets too low (might actually slow things down, change it to larger
partSize = (float)_zRes/stepParts;}
if (partSize < 4) {stepParts = (int)(ceil((float)_zRes/4.0f)); // If it's still too low (only possible on future systems with +24 cores), change it to 4
partSize = (float)_zRes/stepParts;}
#else
int zBegin=0;
int zEnd=_zRes;
#endif
wipeBoundariesSL(0, _zRes);
#if PARALLEL==1
#pragma omp parallel
{
#pragma omp for schedule(static,1)
for (int i=0; i<stepParts; i++)
{
int zBegin = (int)((float)i*partSize + 0.5f);
int zEnd = (int)((float)(i+1)*partSize + 0.5f);
#endif
addVorticity(zBegin, zEnd);
addBuoyancy(_heat, _density, gravity, zBegin, zEnd);
addForce(zBegin, zEnd);
#if PARALLEL==1
} // end of parallel
#pragma omp barrier
#pragma omp single
{
#endif
/*
* addForce() changed Temp values to preserve thread safety
* (previous functions in per thread loop still needed
* original velocity data)
*
* So swap temp values to velocity
*/
SWAP_POINTERS(_xVelocity, _xVelocityTemp);
SWAP_POINTERS(_yVelocity, _yVelocityTemp);
SWAP_POINTERS(_zVelocity, _zVelocityTemp);
#if PARALLEL==1
} // end of single
#pragma omp barrier
#pragma omp for
for (int i=0; i<2; i++)
{
if (i==0)
{
#endif
project();
#if PARALLEL==1
}
else if (i==1)
{
#endif
if (_heat) {
diffuseHeat();
}
#if PARALLEL==1
}
}
#pragma omp barrier
#pragma omp single
{
#endif
/*
* For thread safety use "Old" to read
* "current" values but still allow changing values.
*/
SWAP_POINTERS(_xVelocity, _xVelocityOld);
SWAP_POINTERS(_yVelocity, _yVelocityOld);
SWAP_POINTERS(_zVelocity, _zVelocityOld);
SWAP_POINTERS(_density, _densityOld);
SWAP_POINTERS(_heat, _heatOld);
SWAP_POINTERS(_fuel, _fuelOld);
SWAP_POINTERS(_react, _reactOld);
SWAP_POINTERS(_color_r, _color_rOld);
SWAP_POINTERS(_color_g, _color_gOld);
SWAP_POINTERS(_color_b, _color_bOld);
advectMacCormackBegin(0, _zRes);
#if PARALLEL==1
} // end of single
#pragma omp barrier
#pragma omp for schedule(static,1)
for (int i=0; i<stepParts; i++)
{
int zBegin = (int)((float)i*partSize + 0.5f);
int zEnd = (int)((float)(i+1)*partSize + 0.5f);
#endif
advectMacCormackEnd1(zBegin, zEnd);
#if PARALLEL==1
} // end of parallel
#pragma omp barrier
#pragma omp for schedule(static,1)
for (int i=0; i<stepParts; i++)
{
int zBegin = (int)((float)i*partSize + 0.5f);
int zEnd = (int)((float)(i+1)*partSize + 0.5f);
#endif
advectMacCormackEnd2(zBegin, zEnd);
artificialDampingSL(zBegin, zEnd);
// Using forces as temp arrays
#if PARALLEL==1
}
}
for (int i=1; i<stepParts; i++)
{
int zPos=(int)((float)i*partSize + 0.5f);
artificialDampingExactSL(zPos);
}
#endif
/*
* swap final velocity back to Velocity array
* from temp xForce storage
*/
SWAP_POINTERS(_xVelocity, _xForce);
SWAP_POINTERS(_yVelocity, _yForce);
SWAP_POINTERS(_zVelocity, _zForce);
_totalTime += _dt;
_totalSteps++;
for (int i = 0; i < _totalCells; i++)
{
_xForce[i] = _yForce[i] = _zForce[i] = 0.0f;
}
}
// Set border collision model from RNA setting
void FLUID_3D::setBorderCollisions() {
_colloPrev = *_borderColli; // saving the current value
// boundary conditions of the fluid domain
if (_colloPrev == 0)
{
// No collisions
_domainBcFront = false;
_domainBcTop = false;
_domainBcLeft = false;
}
else if (_colloPrev == 2)
{
// Collide with all sides
_domainBcFront = true;
_domainBcTop = true;
_domainBcLeft = true;
}
else
{
// Default values: Collide with "walls", but not top and bottom
_domainBcFront = true;
_domainBcTop = false;
_domainBcLeft = true;
}
_domainBcBack = _domainBcFront;
_domainBcBottom = _domainBcTop;
_domainBcRight = _domainBcLeft;
// set side obstacles
setBorderObstacles();
}
//////////////////////////////////////////////////////////////////////
// helper function to dampen co-located grid artifacts of given arrays in intervals
// (only needed for velocity, strength (w) depends on testcase...
//////////////////////////////////////////////////////////////////////
void FLUID_3D::artificialDampingSL(int zBegin, int zEnd) {
const float w = 0.9;
memmove(_xForce+(_slabSize*zBegin), _xVelocityTemp+(_slabSize*zBegin), sizeof(float)*_slabSize*(zEnd-zBegin));
memmove(_yForce+(_slabSize*zBegin), _yVelocityTemp+(_slabSize*zBegin), sizeof(float)*_slabSize*(zEnd-zBegin));
memmove(_zForce+(_slabSize*zBegin), _zVelocityTemp+(_slabSize*zBegin), sizeof(float)*_slabSize*(zEnd-zBegin));
if(_totalSteps % 4 == 1) {
for (int z = zBegin+1; z < zEnd-1; z++)
for (int y = 1; y < _res[1]-1; y++)
for (int x = 1+(y+z)%2; x < _res[0]-1; x+=2) {
const int index = x + y*_res[0] + z * _slabSize;
_xForce[index] = (1-w)*_xVelocityTemp[index] + 1.0f/6.0f * w*(
_xVelocityTemp[index+1] + _xVelocityTemp[index-1] +
_xVelocityTemp[index+_res[0]] + _xVelocityTemp[index-_res[0]] +
_xVelocityTemp[index+_slabSize] + _xVelocityTemp[index-_slabSize] );
_yForce[index] = (1-w)*_yVelocityTemp[index] + 1.0f/6.0f * w*(
_yVelocityTemp[index+1] + _yVelocityTemp[index-1] +
_yVelocityTemp[index+_res[0]] + _yVelocityTemp[index-_res[0]] +
_yVelocityTemp[index+_slabSize] + _yVelocityTemp[index-_slabSize] );
_zForce[index] = (1-w)*_zVelocityTemp[index] + 1.0f/6.0f * w*(
_zVelocityTemp[index+1] + _zVelocityTemp[index-1] +
_zVelocityTemp[index+_res[0]] + _zVelocityTemp[index-_res[0]] +
_zVelocityTemp[index+_slabSize] + _zVelocityTemp[index-_slabSize] );
}
}
if(_totalSteps % 4 == 3) {
for (int z = zBegin+1; z < zEnd-1; z++)
for (int y = 1; y < _res[1]-1; y++)
for (int x = 1+(y+z+1)%2; x < _res[0]-1; x+=2) {
const int index = x + y*_res[0] + z * _slabSize;
_xForce[index] = (1-w)*_xVelocityTemp[index] + 1.0f/6.0f * w*(
_xVelocityTemp[index+1] + _xVelocityTemp[index-1] +
_xVelocityTemp[index+_res[0]] + _xVelocityTemp[index-_res[0]] +
_xVelocityTemp[index+_slabSize] + _xVelocityTemp[index-_slabSize] );
_yForce[index] = (1-w)*_yVelocityTemp[index] + 1.0f/6.0f * w*(
_yVelocityTemp[index+1] + _yVelocityTemp[index-1] +
_yVelocityTemp[index+_res[0]] + _yVelocityTemp[index-_res[0]] +
_yVelocityTemp[index+_slabSize] + _yVelocityTemp[index-_slabSize] );
_zForce[index] = (1-w)*_zVelocityTemp[index] + 1.0f/6.0f * w*(
_zVelocityTemp[index+1] + _zVelocityTemp[index-1] +
_zVelocityTemp[index+_res[0]] + _zVelocityTemp[index-_res[0]] +
_zVelocityTemp[index+_slabSize] + _zVelocityTemp[index-_slabSize] );
}
}
}
void FLUID_3D::artificialDampingExactSL(int pos) {
const float w = 0.9;
int index, x,y,z;
size_t posslab;
for (z=pos-1; z<=pos; z++)
{
posslab=z * _slabSize;
if(_totalSteps % 4 == 1) {
for (y = 1; y < _res[1]-1; y++)
for (x = 1+(y+z)%2; x < _res[0]-1; x+=2) {
index = x + y*_res[0] + posslab;
/*
* Uses xForce as temporary storage to allow other threads to read
* old values from xVelocityTemp
*/
_xForce[index] = (1-w)*_xVelocityTemp[index] + 1.0f/6.0f * w*(
_xVelocityTemp[index+1] + _xVelocityTemp[index-1] +
_xVelocityTemp[index+_res[0]] + _xVelocityTemp[index-_res[0]] +
_xVelocityTemp[index+_slabSize] + _xVelocityTemp[index-_slabSize] );
_yForce[index] = (1-w)*_yVelocityTemp[index] + 1.0f/6.0f * w*(
_yVelocityTemp[index+1] + _yVelocityTemp[index-1] +
_yVelocityTemp[index+_res[0]] + _yVelocityTemp[index-_res[0]] +
_yVelocityTemp[index+_slabSize] + _yVelocityTemp[index-_slabSize] );
_zForce[index] = (1-w)*_zVelocityTemp[index] + 1.0f/6.0f * w*(
_zVelocityTemp[index+1] + _zVelocityTemp[index-1] +
_zVelocityTemp[index+_res[0]] + _zVelocityTemp[index-_res[0]] +
_zVelocityTemp[index+_slabSize] + _zVelocityTemp[index-_slabSize] );
}
}
if(_totalSteps % 4 == 3) {
for (y = 1; y < _res[1]-1; y++)
for (x = 1+(y+z+1)%2; x < _res[0]-1; x+=2) {
index = x + y*_res[0] + posslab;
/*
* Uses xForce as temporary storage to allow other threads to read
* old values from xVelocityTemp
*/
_xForce[index] = (1-w)*_xVelocityTemp[index] + 1.0f/6.0f * w*(
_xVelocityTemp[index+1] + _xVelocityTemp[index-1] +
_xVelocityTemp[index+_res[0]] + _xVelocityTemp[index-_res[0]] +
_xVelocityTemp[index+_slabSize] + _xVelocityTemp[index-_slabSize] );
_yForce[index] = (1-w)*_yVelocityTemp[index] + 1.0f/6.0f * w*(
_yVelocityTemp[index+1] + _yVelocityTemp[index-1] +
_yVelocityTemp[index+_res[0]] + _yVelocityTemp[index-_res[0]] +
_yVelocityTemp[index+_slabSize] + _yVelocityTemp[index-_slabSize] );
_zForce[index] = (1-w)*_zVelocityTemp[index] + 1.0f/6.0f * w*(
_zVelocityTemp[index+1] + _zVelocityTemp[index-1] +
_zVelocityTemp[index+_res[0]] + _zVelocityTemp[index-_res[0]] +
_zVelocityTemp[index+_slabSize] + _zVelocityTemp[index-_slabSize] );
}
}
}
}
//////////////////////////////////////////////////////////////////////
// copy out the boundary in all directions
//////////////////////////////////////////////////////////////////////
void FLUID_3D::copyBorderAll(float* field, int zBegin, int zEnd)
{
int index, x, y, z;
int zSize = zEnd-zBegin;
int _blockTotalCells=_slabSize * zSize;
if (zBegin==0)
for (int y = 0; y < _yRes; y++)
for (int x = 0; x < _xRes; x++)
{
// front slab
index = x + y * _xRes;
field[index] = field[index + _slabSize];
}
if (zEnd==_zRes)
for (y = 0; y < _yRes; y++)
for (x = 0; x < _xRes; x++)
{
// back slab
index = x + y * _xRes + _blockTotalCells - _slabSize;
field[index] = field[index - _slabSize];
}
for (z = 0; z < zSize; z++)
for (x = 0; x < _xRes; x++)
{
// bottom slab
index = x + z * _slabSize;
field[index] = field[index + _xRes];
// top slab
index += _slabSize - _xRes;
field[index] = field[index - _xRes];
}
for (z = 0; z < zSize; z++)
for (y = 0; y < _yRes; y++)
{
// left slab
index = y * _xRes + z * _slabSize;
field[index] = field[index + 1];
// right slab
index += _xRes - 1;
field[index] = field[index - 1];
}
}
//////////////////////////////////////////////////////////////////////
// wipe boundaries of velocity and density
//////////////////////////////////////////////////////////////////////
void FLUID_3D::wipeBoundaries(int zBegin, int zEnd)
{
setZeroBorder(_xVelocity, _res, zBegin, zEnd);
setZeroBorder(_yVelocity, _res, zBegin, zEnd);
setZeroBorder(_zVelocity, _res, zBegin, zEnd);
setZeroBorder(_density, _res, zBegin, zEnd);
if (_fuel) {
setZeroBorder(_fuel, _res, zBegin, zEnd);
setZeroBorder(_react, _res, zBegin, zEnd);
}
if (_color_r) {
setZeroBorder(_color_r, _res, zBegin, zEnd);
setZeroBorder(_color_g, _res, zBegin, zEnd);
setZeroBorder(_color_b, _res, zBegin, zEnd);
}
}
void FLUID_3D::wipeBoundariesSL(int zBegin, int zEnd)
{
/////////////////////////////////////
// setZeroBorder to all:
/////////////////////////////////////
/////////////////////////////////////
// setZeroX
/////////////////////////////////////
const int slabSize = _xRes * _yRes;
int index, x,y,z;
for (z = zBegin; z < zEnd; z++)
for (y = 0; y < _yRes; y++)
{
// left slab
index = y * _xRes + z * slabSize;
_xVelocity[index] = 0.0f;
_yVelocity[index] = 0.0f;
_zVelocity[index] = 0.0f;
_density[index] = 0.0f;
if (_fuel) {
_fuel[index] = 0.0f;
_react[index] = 0.0f;
}
if (_color_r) {
_color_r[index] = 0.0f;
_color_g[index] = 0.0f;
_color_b[index] = 0.0f;
}
// right slab
index += _xRes - 1;
_xVelocity[index] = 0.0f;
_yVelocity[index] = 0.0f;
_zVelocity[index] = 0.0f;
_density[index] = 0.0f;
if (_fuel) {
_fuel[index] = 0.0f;
_react[index] = 0.0f;
}
if (_color_r) {
_color_r[index] = 0.0f;
_color_g[index] = 0.0f;
_color_b[index] = 0.0f;
}
}
/////////////////////////////////////
// setZeroY
/////////////////////////////////////
for (z = zBegin; z < zEnd; z++)
for (x = 0; x < _xRes; x++)
{
// bottom slab
index = x + z * slabSize;
_xVelocity[index] = 0.0f;
_yVelocity[index] = 0.0f;
_zVelocity[index] = 0.0f;
_density[index] = 0.0f;
if (_fuel) {
_fuel[index] = 0.0f;
_react[index] = 0.0f;
}
if (_color_r) {
_color_r[index] = 0.0f;
_color_g[index] = 0.0f;
_color_b[index] = 0.0f;
}
// top slab
index += slabSize - _xRes;
_xVelocity[index] = 0.0f;
_yVelocity[index] = 0.0f;
_zVelocity[index] = 0.0f;
_density[index] = 0.0f;
if (_fuel) {
_fuel[index] = 0.0f;
_react[index] = 0.0f;
}
if (_color_r) {
_color_r[index] = 0.0f;
_color_g[index] = 0.0f;
_color_b[index] = 0.0f;
}
}
/////////////////////////////////////
// setZeroZ
/////////////////////////////////////
const int totalCells = _xRes * _yRes * _zRes;
index = 0;
if (zBegin == 0)
for (y = 0; y < _yRes; y++)
for (x = 0; x < _xRes; x++, index++)
{
// front slab
_xVelocity[index] = 0.0f;
_yVelocity[index] = 0.0f;
_zVelocity[index] = 0.0f;
_density[index] = 0.0f;
if (_fuel) {
_fuel[index] = 0.0f;
_react[index] = 0.0f;
}
if (_color_r) {
_color_r[index] = 0.0f;
_color_g[index] = 0.0f;
_color_b[index] = 0.0f;
}
}
if (zEnd == _zRes)
{
index=0;
int index_top=0;
const int cellsslab = totalCells - slabSize;
for (y = 0; y < _yRes; y++)
for (x = 0; x < _xRes; x++, index++)
{
// back slab
index_top = index + cellsslab;
_xVelocity[index_top] = 0.0f;
_yVelocity[index_top] = 0.0f;
_zVelocity[index_top] = 0.0f;
_density[index_top] = 0.0f;
if (_fuel) {
_fuel[index_top] = 0.0f;
_react[index_top] = 0.0f;
}
if (_color_r) {
_color_r[index_top] = 0.0f;
_color_g[index_top] = 0.0f;
_color_b[index_top] = 0.0f;
}
}
}
}
//////////////////////////////////////////////////////////////////////
// add forces to velocity field
//////////////////////////////////////////////////////////////////////
void FLUID_3D::addForce(int zBegin, int zEnd)
{
int begin=zBegin * _slabSize;
int end=begin + (zEnd - zBegin) * _slabSize;
for (int i = begin; i < end; i++)
{
_xVelocityTemp[i] = _xVelocity[i] + _dt * _xForce[i];
_yVelocityTemp[i] = _yVelocity[i] + _dt * _yForce[i];
_zVelocityTemp[i] = _zVelocity[i] + _dt * _zForce[i];
}
}
//////////////////////////////////////////////////////////////////////
// project into divergence free field
//////////////////////////////////////////////////////////////////////
void FLUID_3D::project()
{
int x, y, z;
size_t index;
float *_pressure = new float[_totalCells];
float *_divergence = new float[_totalCells];
memset(_pressure, 0, sizeof(float)*_totalCells);
memset(_divergence, 0, sizeof(float)*_totalCells);
// set velocity and pressure inside of obstacles to zero
setObstacleBoundaries(_pressure, 0, _zRes);
// copy out the boundaries
if(!_domainBcLeft) setNeumannX(_xVelocity, _res, 0, _zRes);
else setZeroX(_xVelocity, _res, 0, _zRes);
if(!_domainBcFront) setNeumannY(_yVelocity, _res, 0, _zRes);
else setZeroY(_yVelocity, _res, 0, _zRes);
if(!_domainBcTop) setNeumannZ(_zVelocity, _res, 0, _zRes);
else setZeroZ(_zVelocity, _res, 0, _zRes);
// calculate divergence
index = _slabSize + _xRes + 1;
for (z = 1; z < _zRes - 1; z++, index += 2 * _xRes)
for (y = 1; y < _yRes - 1; y++, index += 2)
for (x = 1; x < _xRes - 1; x++, index++)
{
if(_obstacles[index])
{
_divergence[index] = 0.0f;
continue;
}
float xright = _xVelocity[index + 1];
float xleft = _xVelocity[index - 1];
float yup = _yVelocity[index + _xRes];
float ydown = _yVelocity[index - _xRes];
float ztop = _zVelocity[index + _slabSize];
float zbottom = _zVelocity[index - _slabSize];
if(_obstacles[index+1]) xright = - _xVelocity[index]; // DG: +=
if(_obstacles[index-1]) xleft = - _xVelocity[index];
if(_obstacles[index+_xRes]) yup = - _yVelocity[index];
if(_obstacles[index-_xRes]) ydown = - _yVelocity[index];
if(_obstacles[index+_slabSize]) ztop = - _zVelocity[index];
if(_obstacles[index-_slabSize]) zbottom = - _zVelocity[index];
if(_obstacles[index+1] & 8) xright += _xVelocityOb[index + 1];
if(_obstacles[index-1] & 8) xleft += _xVelocityOb[index - 1];
if(_obstacles[index+_xRes] & 8) yup += _yVelocityOb[index + _xRes];
if(_obstacles[index-_xRes] & 8) ydown += _yVelocityOb[index - _xRes];
if(_obstacles[index+_slabSize] & 8) ztop += _zVelocityOb[index + _slabSize];
if(_obstacles[index-_slabSize] & 8) zbottom += _zVelocityOb[index - _slabSize];
_divergence[index] = -_dx * 0.5f * (
xright - xleft +
yup - ydown +
ztop - zbottom );
// Pressure is zero anyway since now a local array is used
_pressure[index] = 0.0f;
}
copyBorderAll(_pressure, 0, _zRes);
// fix fluid compression caused in isolated components by obstacle movement
fixObstacleCompression(_divergence);
// solve Poisson equation
solvePressurePre(_pressure, _divergence, _obstacles);
setObstaclePressure(_pressure, 0, _zRes);
// project out solution
// New idea for code from NVIDIA graphic gems 3 - DG
float invDx = 1.0f / _dx;
index = _slabSize + _xRes + 1;
for (z = 1; z < _zRes - 1; z++, index += 2 * _xRes)
for (y = 1; y < _yRes - 1; y++, index += 2)
for (x = 1; x < _xRes - 1; x++, index++)
{
float vMask[3] = {1.0f, 1.0f, 1.0f}, vObst[3] = {0, 0, 0};
// float vR = 0.0f, vL = 0.0f, vT = 0.0f, vB = 0.0f, vD = 0.0f, vU = 0.0f; // UNUSED
float pC = _pressure[index]; // center
float pR = _pressure[index + 1]; // right
float pL = _pressure[index - 1]; // left
float pU = _pressure[index + _xRes]; // Up
float pD = _pressure[index - _xRes]; // Down
float pT = _pressure[index + _slabSize]; // top
float pB = _pressure[index - _slabSize]; // bottom
if(!_obstacles[index])
{
// DG TODO: What if obstacle is left + right and one of them is moving?
if(_obstacles[index+1]) { pR = pC; vObst[0] = _xVelocityOb[index + 1]; vMask[0] = 0; }
if(_obstacles[index-1]) { pL = pC; vObst[0] = _xVelocityOb[index - 1]; vMask[0] = 0; }
if(_obstacles[index+_xRes]) { pU = pC; vObst[1] = _yVelocityOb[index + _xRes]; vMask[1] = 0; }
if(_obstacles[index-_xRes]) { pD = pC; vObst[1] = _yVelocityOb[index - _xRes]; vMask[1] = 0; }
if(_obstacles[index+_slabSize]) { pT = pC; vObst[2] = _zVelocityOb[index + _slabSize]; vMask[2] = 0; }
if(_obstacles[index-_slabSize]) { pB = pC; vObst[2] = _zVelocityOb[index - _slabSize]; vMask[2] = 0; }
_xVelocity[index] -= 0.5f * (pR - pL) * invDx;
_yVelocity[index] -= 0.5f * (pU - pD) * invDx;
_zVelocity[index] -= 0.5f * (pT - pB) * invDx;
_xVelocity[index] = (vMask[0] * _xVelocity[index]) + vObst[0];
_yVelocity[index] = (vMask[1] * _yVelocity[index]) + vObst[1];
_zVelocity[index] = (vMask[2] * _zVelocity[index]) + vObst[2];
}
else
{
_xVelocity[index] = _xVelocityOb[index];
_yVelocity[index] = _yVelocityOb[index];
_zVelocity[index] = _zVelocityOb[index];
}
}
// DG: was enabled in original code but now we do this later
// setObstacleVelocity(0, _zRes);
if (_pressure) delete[] _pressure;
if (_divergence) delete[] _divergence;
}
//////////////////////////////////////////////////////////////////////
// calculate the obstacle velocity at boundary
//////////////////////////////////////////////////////////////////////
void FLUID_3D::setObstacleVelocity(int zBegin, int zEnd)
{
// completely TODO <-- who wrote this and what is here TODO? DG
const size_t index_ = _slabSize + _xRes + 1;
//int vIndex=_slabSize + _xRes + 1;
int bb=0;
int bt=0;
if (zBegin == 0) {bb = 1;}
if (zEnd == _zRes) {bt = 1;}
// tag remaining obstacle blocks
for (int z = zBegin + bb; z < zEnd - bt; z++)
{
size_t index = index_ +(z-1)*_slabSize;
for (int y = 1; y < _yRes - 1; y++, index += 2)
{
for (int x = 1; x < _xRes - 1; x++, index++)
{
if (!_obstacles[index])
{
// if(_obstacles[index+1]) xright = - _xVelocityOb[index];
if((_obstacles[index - 1] & 8) && abs(_xVelocityOb[index - 1]) > FLT_EPSILON )
{
// printf("velocity x!\n");
_xVelocity[index] = _xVelocityOb[index - 1];
_xVelocity[index - 1] = _xVelocityOb[index - 1];
}
// if(_obstacles[index+_xRes]) yup = - _yVelocityOb[index];
if((_obstacles[index - _xRes] & 8) && abs(_yVelocityOb[index - _xRes]) > FLT_EPSILON)
{
// printf("velocity y!\n");
_yVelocity[index] = _yVelocityOb[index - _xRes];
_yVelocity[index - _xRes] = _yVelocityOb[index - _xRes];
}
// if(_obstacles[index+_slabSize]) ztop = - _zVelocityOb[index];
if((_obstacles[index - _slabSize] & 8) && abs(_zVelocityOb[index - _slabSize]) > FLT_EPSILON)
{
// printf("velocity z!\n");
_zVelocity[index] = _zVelocityOb[index - _slabSize];
_zVelocity[index - _slabSize] = _zVelocityOb[index - _slabSize];
}
}
else
{
_density[index] = 0;
}
//vIndex++;
} // x loop
//vIndex += 2;
} // y loop
//vIndex += 2 * _xRes;
} // z loop
}
//////////////////////////////////////////////////////////////////////
// diffuse heat
//////////////////////////////////////////////////////////////////////
void FLUID_3D::diffuseHeat()
{
SWAP_POINTERS(_heat, _heatOld);
copyBorderAll(_heatOld, 0, _zRes);
solveHeat(_heat, _heatOld, _obstacles);
// zero out inside obstacles
for (int x = 0; x < _totalCells; x++)
if (_obstacles[x])
_heat[x] = 0.0f;
}
//////////////////////////////////////////////////////////////////////
// stamp an obstacle in the _obstacles field
//////////////////////////////////////////////////////////////////////
void FLUID_3D::addObstacle(OBSTACLE* obstacle)
{
int index = 0;
for (int z = 0; z < _zRes; z++)
for (int y = 0; y < _yRes; y++)
for (int x = 0; x < _xRes; x++, index++)
if (obstacle->inside(x * _dx, y * _dx, z * _dx)) {
_obstacles[index] = true;
}
}
//////////////////////////////////////////////////////////////////////
// calculate the obstacle directional types
//////////////////////////////////////////////////////////////////////
void FLUID_3D::setObstaclePressure(float *_pressure, int zBegin, int zEnd)
{
// completely TODO <-- who wrote this and what is here TODO? DG
const size_t index_ = _slabSize + _xRes + 1;
//int vIndex=_slabSize + _xRes + 1;
int bb=0;
int bt=0;
if (zBegin == 0) {bb = 1;}
if (zEnd == _zRes) {bt = 1;}
// tag remaining obstacle blocks
for (int z = zBegin + bb; z < zEnd - bt; z++)
{
size_t index = index_ +(z-1)*_slabSize;
for (int y = 1; y < _yRes - 1; y++, index += 2)
{
for (int x = 1; x < _xRes - 1; x++, index++)
{
// could do cascade of ifs, but they are a pain
if (_obstacles[index] /* && !(_obstacles[index] & 8) DG TODO TEST THIS CONDITION */)
{
const int top = _obstacles[index + _slabSize];
const int bottom= _obstacles[index - _slabSize];
const int up = _obstacles[index + _xRes];
const int down = _obstacles[index - _xRes];
const int left = _obstacles[index - 1];
const int right = _obstacles[index + 1];
// unused
// const bool fullz = (top && bottom);
// const bool fully = (up && down);
//const bool fullx = (left && right);
/*
_xVelocity[index] =
_yVelocity[index] =
_zVelocity[index] = 0.0f;
*/
_pressure[index] = 0.0f;
// average pressure neighbors
float pcnt = 0.;
if (left && !right) {
_pressure[index] += _pressure[index + 1];
pcnt += 1.0f;
}
if (!left && right) {
_pressure[index] += _pressure[index - 1];
pcnt += 1.0f;
}
if (up && !down) {
_pressure[index] += _pressure[index - _xRes];
pcnt += 1.0f;
}
if (!up && down) {
_pressure[index] += _pressure[index + _xRes];
pcnt += 1.0f;
}
if (top && !bottom) {
_pressure[index] += _pressure[index - _slabSize];
pcnt += 1.0f;
}
if (!top && bottom) {
_pressure[index] += _pressure[index + _slabSize];
pcnt += 1.0f;
}
if(pcnt > 0.000001f)
_pressure[index] /= pcnt;
// TODO? set correct velocity bc's
// velocities are only set to zero right now
// this means it's not a full no-slip boundary condition
// but a "half-slip" - still looks ok right now
}
//vIndex++;
} // x loop
//vIndex += 2;
} // y loop
//vIndex += 2 * _xRes;
} // z loop
}
void FLUID_3D::setObstacleBoundaries(float *_pressure, int zBegin, int zEnd)
{
// cull degenerate obstacles , move to addObstacle?
// r = b - Ax
const size_t index_ = _slabSize + _xRes + 1;
int bb=0;
int bt=0;
if (zBegin == 0) {bb = 1;}
if (zEnd == _zRes) {bt = 1;}
for (int z = zBegin + bb; z < zEnd - bt; z++)
{
size_t index = index_ +(z-1)*_slabSize;
for (int y = 1; y < _yRes - 1; y++, index += 2)
{
for (int x = 1; x < _xRes - 1; x++, index++)
{
if (_obstacles[index] != EMPTY)
{
const int top = _obstacles[index + _slabSize];
const int bottom= _obstacles[index - _slabSize];
const int up = _obstacles[index + _xRes];
const int down = _obstacles[index - _xRes];
const int left = _obstacles[index - 1];
const int right = _obstacles[index + 1];
int counter = 0;
if (up) counter++;
if (down) counter++;
if (left) counter++;
if (right) counter++;
if (top) counter++;
if (bottom) counter++;
if (counter < 3)
_obstacles[index] = EMPTY;
}
if (_obstacles[index])
{
_xVelocity[index] =
_yVelocity[index] =
_zVelocity[index] = 0.0f;
_pressure[index] = 0.0f;
}
//vIndex++;
} // x-loop
//vIndex += 2;
} // y-loop
//vIndex += 2* _xRes;
} // z-loop
}
void FLUID_3D::floodFillComponent(int *buffer, size_t *queue, size_t limit, size_t pos, int from, int to)
{
/* Flood 'from' cells with 'to' in the grid. Rely on (from != 0 && from != to && edges == 0) to stop. */
int offsets[] = { -1, +1, -_xRes, +_xRes, -_slabSize, +_slabSize };
size_t qend = 0;
buffer[pos] = to;
queue[qend++] = pos;
for (size_t qidx = 0; qidx < qend; qidx++)
{
pos = queue[qidx];
for (int i = 0; i < 6; i++)
{
size_t next = pos + offsets[i];
if (next < limit && buffer[next] == from)
{
buffer[next] = to;
queue[qend++] = next;
}
}
}
}
void FLUID_3D::mergeComponents(int *buffer, size_t *queue, size_t cur, size_t other)
{
/* Replace higher value with lower. */
if (buffer[other] < buffer[cur])
{
floodFillComponent(buffer, queue, cur, cur, buffer[cur], buffer[other]);
}
else if (buffer[cur] < buffer[other])
{
floodFillComponent(buffer, queue, cur, other, buffer[other], buffer[cur]);
}
}
void FLUID_3D::fixObstacleCompression(float *divergence)
{
int x, y, z;
size_t index;
/* Find compartments completely separated by obstacles.
* Edge of the domain is automatically component 0. */
int *component = new int[_totalCells];
size_t *queue = new size_t[_totalCells];
memset(component, 0, sizeof(int) * _totalCells);
int next_id = 1;
for (z = 1, index = _slabSize + _xRes + 1; z < _zRes - 1; z++, index += 2 * _xRes)
{
for (y = 1; y < _yRes - 1; y++, index += 2)
{
for (x = 1; x < _xRes - 1; x++, index++)
{
if(!_obstacles[index])
{
/* Check for connection to the domain edge at iteration end. */
if ((x == _xRes-2 && !_obstacles[index + 1]) ||
(y == _yRes-2 && !_obstacles[index + _xRes]) ||
(z == _zRes-2 && !_obstacles[index + _slabSize]))
{
component[index] = 0;
}
else {
component[index] = next_id;
}
if (!_obstacles[index - 1])
mergeComponents(component, queue, index, index - 1);
if (!_obstacles[index - _xRes])
mergeComponents(component, queue, index, index - _xRes);
if (!_obstacles[index - _slabSize])
mergeComponents(component, queue, index, index - _slabSize);
if (component[index] == next_id)
next_id++;
}
}
}
}
delete[] queue;
/* Compute average divergence within each component. */
float *total_divergence = new float[next_id];
int *component_size = new int[next_id];
memset(total_divergence, 0, sizeof(float) * next_id);
memset(component_size, 0, sizeof(int) * next_id);
for (z = 1, index = _slabSize + _xRes + 1; z < _zRes - 1; z++, index += 2 * _xRes)
{
for (y = 1; y < _yRes - 1; y++, index += 2)
{
for (x = 1; x < _xRes - 1; x++, index++)
{
if(!_obstacles[index])
{
int ci = component[index];
component_size[ci]++;
total_divergence[ci] += divergence[index];
}
}
}
}
/* Adjust divergence to make the average zero in each component except the edge. */
total_divergence[0] = 0.0f;
for (z = 1, index = _slabSize + _xRes + 1; z < _zRes - 1; z++, index += 2 * _xRes)
{
for (y = 1; y < _yRes - 1; y++, index += 2)
{
for (x = 1; x < _xRes - 1; x++, index++)
{
if(!_obstacles[index])
{
int ci = component[index];
divergence[index] -= total_divergence[ci] / component_size[ci];
}
}
}
}
delete[] component;
delete[] component_size;
delete[] total_divergence;
}
//////////////////////////////////////////////////////////////////////
// add buoyancy forces
//////////////////////////////////////////////////////////////////////
void FLUID_3D::addBuoyancy(float *heat, float *density, float gravity[3], int zBegin, int zEnd)
{
int index = zBegin*_slabSize;
for (int z = zBegin; z < zEnd; z++)
for (int y = 0; y < _yRes; y++)
for (int x = 0; x < _xRes; x++, index++)
{
float buoyancy = *_alpha * density[index] + (*_beta * (((heat) ? heat[index] : 0.0f) - _tempAmb));
_xForce[index] -= gravity[0] * buoyancy;
_yForce[index] -= gravity[1] * buoyancy;
_zForce[index] -= gravity[2] * buoyancy;
}
}
//////////////////////////////////////////////////////////////////////
// add vorticity to the force field
//////////////////////////////////////////////////////////////////////
#define VORT_VEL(i, j) \
((_obstacles[obpos[(i)]] & 8) ? ((abs(objvelocity[(j)][obpos[(i)]]) > FLT_EPSILON) ? objvelocity[(j)][obpos[(i)]] : velocity[(j)][index]) : velocity[(j)][obpos[(i)]])
void FLUID_3D::addVorticity(int zBegin, int zEnd)
{
// set flame vorticity from RNA value
float flame_vorticity = (*_flame_vorticity)/_constantScaling;
//int x,y,z,index;
if(_vorticityEps+flame_vorticity<=0.0f) return;
int _blockSize=zEnd-zBegin;
int _blockTotalCells = _slabSize * (_blockSize+2);
float *_xVorticity, *_yVorticity, *_zVorticity, *_vorticity;
int bb=0;
int bt=0;
int bb1=-1;
int bt1=-1;
if (zBegin == 0) {bb1 = 1; bb = 1; _blockTotalCells-=_blockSize;}
if (zEnd == _zRes) {bt1 = 1;bt = 1; _blockTotalCells-=_blockSize;}
_xVorticity = new float[_blockTotalCells];
_yVorticity = new float[_blockTotalCells];
_zVorticity = new float[_blockTotalCells];
_vorticity = new float[_blockTotalCells];
memset(_xVorticity, 0, sizeof(float)*_blockTotalCells);
memset(_yVorticity, 0, sizeof(float)*_blockTotalCells);
memset(_zVorticity, 0, sizeof(float)*_blockTotalCells);
memset(_vorticity, 0, sizeof(float)*_blockTotalCells);
//const size_t indexsetupV=_slabSize;
const size_t index_ = _slabSize + _xRes + 1;
// calculate vorticity
float gridSize = 0.5f / _dx;
//index = _slabSize + _xRes + 1;
float *velocity[3];
float *objvelocity[3];
velocity[0] = _xVelocity;
velocity[1] = _yVelocity;
velocity[2] = _zVelocity;
objvelocity[0] = _xVelocityOb;
objvelocity[1] = _yVelocityOb;
objvelocity[2] = _zVelocityOb;
size_t vIndex=_xRes + 1;
for (int z = zBegin + bb1; z < (zEnd - bt1); z++)
{
size_t index = index_ +(z-1)*_slabSize;
vIndex = index-(zBegin-1+bb)*_slabSize;
for (int y = 1; y < _yRes - 1; y++, index += 2)
{
for (int x = 1; x < _xRes - 1; x++, index++)
{
if (!_obstacles[index])
{
int obpos[6];
obpos[0] = (_obstacles[index + _xRes] == 1) ? index : index + _xRes; // up
obpos[1] = (_obstacles[index - _xRes] == 1) ? index : index - _xRes; // down
float dy = (obpos[0] == index || obpos[1] == index) ? 1.0f / _dx : gridSize;
obpos[2] = (_obstacles[index + _slabSize] == 1) ? index : index + _slabSize; // out
obpos[3] = (_obstacles[index - _slabSize] == 1) ? index : index - _slabSize; // in
float dz = (obpos[2] == index || obpos[3] == index) ? 1.0f / _dx : gridSize;
obpos[4] = (_obstacles[index + 1] == 1) ? index : index + 1; // right
obpos[5] = (_obstacles[index - 1] == 1) ? index : index - 1; // left
float dx = (obpos[4] == index || obpos[5] == index) ? 1.0f / _dx : gridSize;
float xV[2], yV[2], zV[2];
zV[1] = VORT_VEL(0, 2);
zV[0] = VORT_VEL(1, 2);
yV[1] = VORT_VEL(2, 1);
yV[0] = VORT_VEL(3, 1);
_xVorticity[vIndex] = (zV[1] - zV[0]) * dy + (-yV[1] + yV[0]) * dz;
xV[1] = VORT_VEL(2, 0);
xV[0] = VORT_VEL(3, 0);
zV[1] = VORT_VEL(4, 2);
zV[0] = VORT_VEL(5, 2);
_yVorticity[vIndex] = (xV[1] - xV[0]) * dz + (-zV[1] + zV[0]) * dx;
yV[1] = VORT_VEL(4, 1);
yV[0] = VORT_VEL(5, 1);
xV[1] = VORT_VEL(0, 0);
xV[0] = VORT_VEL(1, 0);
_zVorticity[vIndex] = (yV[1] - yV[0]) * dx + (-xV[1] + xV[0])* dy;
_vorticity[vIndex] = sqrtf(_xVorticity[vIndex] * _xVorticity[vIndex] +
_yVorticity[vIndex] * _yVorticity[vIndex] +
_zVorticity[vIndex] * _zVorticity[vIndex]);
}
vIndex++;
}
vIndex+=2;
}
//vIndex+=2*_xRes;
}
// calculate normalized vorticity vectors
float eps = _vorticityEps;
//index = _slabSize + _xRes + 1;
vIndex=_slabSize + _xRes + 1;
for (int z = zBegin + bb; z < (zEnd - bt); z++)
{
size_t index = index_ +(z-1)*_slabSize;
vIndex = index-(zBegin-1+bb)*_slabSize;
for (int y = 1; y < _yRes - 1; y++, index += 2)
{
for (int x = 1; x < _xRes - 1; x++, index++)
{
//
if (!_obstacles[index])
{
float N[3];
int up = (_obstacles[index + _xRes] == 1) ? vIndex : vIndex + _xRes;
int down = (_obstacles[index - _xRes] == 1) ? vIndex : vIndex - _xRes;
float dy = (up == vIndex || down == vIndex) ? 1.0f / _dx : gridSize;
int out = (_obstacles[index + _slabSize] == 1) ? vIndex : vIndex + _slabSize;
int in = (_obstacles[index - _slabSize] == 1) ? vIndex : vIndex - _slabSize;
float dz = (out == vIndex || in == vIndex) ? 1.0f / _dx : gridSize;
int right = (_obstacles[index + 1] == 1) ? vIndex : vIndex + 1;
int left = (_obstacles[index - 1] == 1) ? vIndex : vIndex - 1;
float dx = (right == vIndex || left == vIndex) ? 1.0f / _dx : gridSize;
N[0] = (_vorticity[right] - _vorticity[left]) * dx;
N[1] = (_vorticity[up] - _vorticity[down]) * dy;
N[2] = (_vorticity[out] - _vorticity[in]) * dz;
float magnitude = sqrtf(N[0] * N[0] + N[1] * N[1] + N[2] * N[2]);
if (magnitude > FLT_EPSILON)
{
float flame_vort = (_fuel) ? _fuel[index]*flame_vorticity : 0.0f;
magnitude = 1.0f / magnitude;
N[0] *= magnitude;
N[1] *= magnitude;
N[2] *= magnitude;
_xForce[index] += (N[1] * _zVorticity[vIndex] - N[2] * _yVorticity[vIndex]) * _dx * (eps + flame_vort);
_yForce[index] += (N[2] * _xVorticity[vIndex] - N[0] * _zVorticity[vIndex]) * _dx * (eps + flame_vort);
_zForce[index] += (N[0] * _yVorticity[vIndex] - N[1] * _xVorticity[vIndex]) * _dx * (eps + flame_vort);
}
} // if
vIndex++;
} // x loop
vIndex+=2;
} // y loop
//vIndex+=2*_xRes;
} // z loop
if (_xVorticity) delete[] _xVorticity;
if (_yVorticity) delete[] _yVorticity;
if (_zVorticity) delete[] _zVorticity;
if (_vorticity) delete[] _vorticity;
}
void FLUID_3D::advectMacCormackBegin(int zBegin, int zEnd)
{
Vec3Int res = Vec3Int(_xRes,_yRes,_zRes);
setZeroX(_xVelocityOld, res, zBegin, zEnd);
setZeroY(_yVelocityOld, res, zBegin, zEnd);
setZeroZ(_zVelocityOld, res, zBegin, zEnd);
}
//////////////////////////////////////////////////////////////////////
// Advect using the MacCormack method from the Selle paper
//////////////////////////////////////////////////////////////////////
void FLUID_3D::advectMacCormackEnd1(int zBegin, int zEnd)
{
Vec3Int res = Vec3Int(_xRes,_yRes,_zRes);
const float dt0 = _dt / _dx;
int begin=zBegin * _slabSize;
int end=begin + (zEnd - zBegin) * _slabSize;
for (int x = begin; x < end; x++)
_xForce[x] = 0.0;
// advectFieldMacCormack1(dt, xVelocity, yVelocity, zVelocity, oldField, newField, res)
advectFieldMacCormack1(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _densityOld, _densityTemp, res, zBegin, zEnd);
if (_heat) {
advectFieldMacCormack1(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _heatOld, _heatTemp, res, zBegin, zEnd);
}
if (_fuel) {
advectFieldMacCormack1(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _fuelOld, _fuelTemp, res, zBegin, zEnd);
advectFieldMacCormack1(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _reactOld, _reactTemp, res, zBegin, zEnd);
}
if (_color_r) {
advectFieldMacCormack1(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _color_rOld, _color_rTemp, res, zBegin, zEnd);
advectFieldMacCormack1(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _color_gOld, _color_gTemp, res, zBegin, zEnd);
advectFieldMacCormack1(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _color_bOld, _color_bTemp, res, zBegin, zEnd);
}
advectFieldMacCormack1(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _xVelocityOld, _xVelocity, res, zBegin, zEnd);
advectFieldMacCormack1(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _yVelocityOld, _yVelocity, res, zBegin, zEnd);
advectFieldMacCormack1(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _zVelocityOld, _zVelocity, res, zBegin, zEnd);
// Have to wait untill all the threads are done -> so continuing in step 3
}
//////////////////////////////////////////////////////////////////////
// Advect using the MacCormack method from the Selle paper
//////////////////////////////////////////////////////////////////////
void FLUID_3D::advectMacCormackEnd2(int zBegin, int zEnd)
{
const float dt0 = _dt / _dx;
Vec3Int res = Vec3Int(_xRes,_yRes,_zRes);
// use force array as temp array
float* t1 = _xForce;
// advectFieldMacCormack2(dt, xVelocity, yVelocity, zVelocity, oldField, newField, tempfield, temp, res, obstacles)
/* finish advection */
advectFieldMacCormack2(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _densityOld, _density, _densityTemp, t1, res, _obstacles, zBegin, zEnd);
if (_heat) {
advectFieldMacCormack2(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _heatOld, _heat, _heatTemp, t1, res, _obstacles, zBegin, zEnd);
}
if (_fuel) {
advectFieldMacCormack2(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _fuelOld, _fuel, _fuelTemp, t1, res, _obstacles, zBegin, zEnd);
advectFieldMacCormack2(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _reactOld, _react, _reactTemp, t1, res, _obstacles, zBegin, zEnd);
}
if (_color_r) {
advectFieldMacCormack2(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _color_rOld, _color_r, _color_rTemp, t1, res, _obstacles, zBegin, zEnd);
advectFieldMacCormack2(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _color_gOld, _color_g, _color_gTemp, t1, res, _obstacles, zBegin, zEnd);
advectFieldMacCormack2(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _color_bOld, _color_b, _color_bTemp, t1, res, _obstacles, zBegin, zEnd);
}
advectFieldMacCormack2(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _xVelocityOld, _xVelocityTemp, _xVelocity, t1, res, _obstacles, zBegin, zEnd);
advectFieldMacCormack2(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _yVelocityOld, _yVelocityTemp, _yVelocity, t1, res, _obstacles, zBegin, zEnd);
advectFieldMacCormack2(dt0, _xVelocityOld, _yVelocityOld, _zVelocityOld, _zVelocityOld, _zVelocityTemp, _zVelocity, t1, res, _obstacles, zBegin, zEnd);
/* set boundary conditions for velocity */
if(!_domainBcLeft) copyBorderX(_xVelocityTemp, res, zBegin, zEnd);
else setZeroX(_xVelocityTemp, res, zBegin, zEnd);
if(!_domainBcFront) copyBorderY(_yVelocityTemp, res, zBegin, zEnd);
else setZeroY(_yVelocityTemp, res, zBegin, zEnd);
if(!_domainBcTop) copyBorderZ(_zVelocityTemp, res, zBegin, zEnd);
else setZeroZ(_zVelocityTemp, res, zBegin, zEnd);
/* clear data boundaries */
setZeroBorder(_density, res, zBegin, zEnd);
if (_fuel) {
setZeroBorder(_fuel, res, zBegin, zEnd);
setZeroBorder(_react, res, zBegin, zEnd);
}
if (_color_r) {
setZeroBorder(_color_r, res, zBegin, zEnd);
setZeroBorder(_color_g, res, zBegin, zEnd);
setZeroBorder(_color_b, res, zBegin, zEnd);
}
}
void FLUID_3D::processBurn(float *fuel, float *smoke, float *react, float *heat,
float *r, float *g, float *b, int total_cells, float dt)
{
float burning_rate = *_burning_rate;
float flame_smoke = *_flame_smoke;
float ignition_point = *_ignition_temp;
float temp_max = *_max_temp;
for (int index = 0; index < total_cells; index++)
{
float orig_fuel = fuel[index];
float orig_smoke = smoke[index];
float smoke_emit = 0.0f;
float flame = 0.0f;
/* process fuel */
fuel[index] -= burning_rate * dt;
if (fuel[index] < 0.0f) fuel[index] = 0.0f;
/* process reaction coordinate */
if (orig_fuel > FLT_EPSILON) {
react[index] *= fuel[index]/orig_fuel;
flame = pow(react[index], 0.5f);
}
else {
react[index] = 0.0f;
}
/* emit smoke based on fuel burn rate and "flame_smoke" factor */
smoke_emit = (orig_fuel < 1.0f) ? (1.0f - orig_fuel)*0.5f : 0.0f;
smoke_emit = (smoke_emit + 0.5f) * (orig_fuel-fuel[index]) * 0.1f * flame_smoke;
smoke[index] += smoke_emit;
CLAMP(smoke[index], 0.0f, 1.0f);
/* set fluid temperature from the flame temperature profile */
if (heat && flame)
heat[index] = (1.0f - flame)*ignition_point + flame*temp_max;
/* mix new color */
if (r && smoke_emit > FLT_EPSILON) {
float smoke_factor = smoke[index]/(orig_smoke+smoke_emit);
r[index] = (r[index] + _flame_smoke_color[0] * smoke_emit) * smoke_factor;
g[index] = (g[index] + _flame_smoke_color[1] * smoke_emit) * smoke_factor;
b[index] = (b[index] + _flame_smoke_color[2] * smoke_emit) * smoke_factor;
}
}
}
void FLUID_3D::updateFlame(float *react, float *flame, int total_cells)
{
for (int index = 0; index < total_cells; index++)
{
/* model flame temperature curve from the reaction coordinate (fuel)
* TODO: Would probably be best to get rid of whole "flame" data field.
* Currently it's just sqrt mirror of reaction coordinate, and therefore
* basically just waste of memory and disk space...
*/
if (react[index]>0.0f) {
/* do a smooth falloff for rest of the values */
flame[index] = pow(react[index], 0.5f);
}
else
flame[index] = 0.0f;
}
}
| {
"pile_set_name": "Github"
} |
#include <cstdlib>
#include <ctime>
#include <set>
#include "nemo_set.h"
#include "nemo_mutex.h"
#include "nemo_iterator.h"
#include "util.h"
#include "xdebug.h"
using namespace nemo;
Status Nemo::SGetMetaByKey(const std::string& key, SetMeta& meta) {
std::string meta_val, meta_key = EncodeSSizeKey(key);
Status s = set_db_->Get(rocksdb::ReadOptions(), meta_key, &meta_val);
if (!s.ok()) {
return s;
}
meta.DecodeFrom(meta_val);
return Status::OK();
}
Status Nemo::SChecknRecover(const std::string& key) {
RecordLock l(&mutex_set_record_, key);
SetMeta meta;
Status s = SGetMetaByKey(key, meta);
if (!s.ok()) {
return s;
}
// Generate prefix
std::string key_start = EncodeSetKey(key, "");
// Iterater and cout
int field_count = 0;
rocksdb::Iterator *it;
rocksdb::ReadOptions iterate_options;
iterate_options.snapshot = set_db_->GetSnapshot();
iterate_options.fill_cache = false;
it = set_db_->NewIterator(iterate_options);
it->Seek(key_start);
std::string dbkey, dbfield;
while (it->Valid()) {
if ((it->key())[0] != DataType::kSet) {
break;
}
DecodeSetKey(it->key(), &dbkey, &dbfield);
if (dbkey != key) {
break;
}
++field_count;
it->Next();
}
set_db_->ReleaseSnapshot(iterate_options.snapshot);
delete it;
// Compare
if (meta.len == field_count) {
return Status::OK();
}
// Fix if needed
rocksdb::WriteBatch writebatch;
if (IncrSSize(key, (field_count - meta.len), writebatch) == -1) {
return Status::Corruption("fix set meta error");
}
return set_db_->WriteWithOldKeyTTL(rocksdb::WriteOptions(), &(writebatch));
}
Status Nemo::SAdd(const std::string &key, const std::string &member, int64_t *res) {
if (key.size() >= KEY_MAX_LENGTH || key.size() <= 0) {
return Status::InvalidArgument("Invalid key length");
}
Status s;
RecordLock l(&mutex_set_record_, key);
//MutexLock l(&mutex_set_);
rocksdb::WriteBatch writebatch;
std::string set_key = EncodeSetKey(key, member);
std::string val;
s = set_db_->Get(rocksdb::ReadOptions(), set_key, &val);
if (s.IsNotFound()) { // not found
*res = 1;
if (IncrSSize(key, 1, writebatch) < 0) {
return Status::Corruption("incrSSize error");
}
writebatch.Put(set_key, rocksdb::Slice());
} else if (s.ok()) {
*res = 0;
} else {
return Status::Corruption("sadd check member error");
}
s = set_db_->WriteWithOldKeyTTL(rocksdb::WriteOptions(), &(writebatch));
return s;
}
Status Nemo::SAddNoLock(const std::string &key, const std::string &member, int64_t *res) {
Status s;
rocksdb::WriteBatch writebatch;
std::string set_key = EncodeSetKey(key, member);
std::string val;
s = set_db_->Get(rocksdb::ReadOptions(), set_key, &val);
if (s.IsNotFound()) { // not found
*res = 1;
if (IncrSSize(key, 1, writebatch) < 0) {
return Status::Corruption("incrSSize error");
}
writebatch.Put(set_key, rocksdb::Slice());
} else if (s.ok()) {
*res = 0;
} else {
return Status::Corruption("sadd check member error");
}
s = set_db_->WriteWithOldKeyTTL(rocksdb::WriteOptions(), &(writebatch));
return s;
}
Status Nemo::SRem(const std::string &key, const std::string &member, int64_t *res) {
if (key.size() >= KEY_MAX_LENGTH || key.size() <= 0) {
return Status::InvalidArgument("Invalid key length");
}
Status s;
//MutexLock l(&mutex_set_);
RecordLock l(&mutex_set_record_, key);
rocksdb::WriteBatch writebatch;
std::string set_key = EncodeSetKey(key, member);
std::string val;
s = set_db_->Get(rocksdb::ReadOptions(), set_key, &val);
if (s.ok()) {
*res = 1;
if (IncrSSize(key, -1, writebatch) < 0) {
return Status::Corruption("incrSSize error");
}
writebatch.Delete(set_key);
s = set_db_->WriteWithOldKeyTTL(rocksdb::WriteOptions(), &(writebatch));
} else if (s.IsNotFound()) {
*res = 0;
} else {
return Status::Corruption("srem check member error");
}
return s;
}
Status Nemo::SRemNoLock(const std::string &key, const std::string &member, int64_t *res) {
Status s;
rocksdb::WriteBatch writebatch;
std::string set_key = EncodeSetKey(key, member);
std::string val;
s = set_db_->Get(rocksdb::ReadOptions(), set_key, &val);
if (s.ok()) {
*res = 1;
if (IncrSSize(key, -1, writebatch) < 0) {
return Status::Corruption("incrSSize error");
}
writebatch.Delete(set_key);
s = set_db_->WriteWithOldKeyTTL(rocksdb::WriteOptions(), &(writebatch));
} else if (s.IsNotFound()) {
*res = 0;
} else {
return Status::Corruption("srem check member error");
}
return s;
}
int Nemo::IncrSSize(const std::string &key, int64_t incr, rocksdb::WriteBatch &writebatch) {
int64_t len = SCard(key);
if (len == -1) {
return -1;
}
std::string size_key = EncodeSSizeKey(key);
len += incr;
writebatch.Put(size_key, rocksdb::Slice((char *)&len, sizeof(int64_t)));
// if (len == 0) {
// writebatch.Delete(size_key);
// } else {
// writebatch.Put(size_key, rocksdb::Slice((char *)&len, sizeof(int64_t)));
// }
return 0;
}
int64_t Nemo::SCard(const std::string &key) {
std::string size_key = EncodeSSizeKey(key);
std::string val;
Status s;
s = set_db_->Get(rocksdb::ReadOptions(), size_key, &val);
if (s.IsNotFound()) {
return 0;
} else if(!s.ok()) {
return -1;
} else {
if (val.size() != sizeof(int64_t)) {
return 0;
}
int64_t ret = *(int64_t *)val.data();
return ret < 0 ? 0 : ret;
}
}
SIterator* Nemo::SScan(const std::string &key, uint64_t limit, bool use_snapshot) {
std::string set_key = EncodeSetKey(key, "");
rocksdb::ReadOptions read_options;
if (use_snapshot) {
read_options.snapshot = set_db_->GetSnapshot();
}
read_options.fill_cache = false;
rocksdb::Iterator *it = set_db_->NewIterator(read_options);
it->Seek(set_key);
IteratorOptions iter_options("", limit, read_options);
return new SIterator(it, iter_options, key);
}
Status Nemo::SMembers(const std::string &key, std::vector<std::string> &members) {
SIterator *iter = SScan(key, -1, true);
for (; iter->Valid(); iter->Next()) {
members.push_back(iter->member());
}
set_db_->ReleaseSnapshot(iter->read_options().snapshot);
delete iter;
return Status::OK();
}
Status Nemo::SUnion(const std::vector<std::string> &keys, std::vector<std::string>& members) {
std::map<std::string, bool> result_flag;
for (int i = 0; i < (int)keys.size(); i++) {
RecordLock l(&mutex_set_record_, keys[i]);
SIterator *iter = SScan(keys[i], -1, true);
for (; iter->Valid(); iter->Next()) {
std::string member = iter->member();
if (result_flag.find(member) == result_flag.end()) {
members.push_back(member);
result_flag[member] = 1;
}
}
set_db_->ReleaseSnapshot(iter->read_options().snapshot);
delete iter;
}
return Status::OK();
}
Status Nemo::SUnionStore(const std::string &destination, const std::vector<std::string> &keys, int64_t *res) {
int numkey = keys.size();
//MutexLock l(&mutex_set_);
if (numkey <= 0) {
return Status::InvalidArgument("invalid parameter, no keys");
}
std::map<std::string, int> member_result;
std::map<std::string, int>::iterator it;
for (int i = 0; i < numkey; i++) {
RecordLock l(&mutex_set_record_, keys[i]);
SIterator *iter = SScan(keys[i], -1, true);
for (; iter->Valid(); iter->Next()) {
member_result[iter->member()] = 1;
}
set_db_->ReleaseSnapshot(iter->read_options().snapshot);
delete iter;
}
// we delete the destination if it exists
Status s;
int64_t tmp_res;
RecordLock l(&mutex_set_record_, destination);
if (SCard(destination) > 0) {
SIterator *iter = SScan(destination, -1, true);
for (; iter->Valid(); iter->Next()) {
s = SRemNoLock(destination, iter->member(), &tmp_res);
if (!s.ok()) {
delete iter;
return s;
}
}
set_db_->ReleaseSnapshot(iter->read_options().snapshot);
delete iter;
}
for (it = member_result.begin(); it != member_result.end(); it++) {
s = SAddNoLock(destination, it->first, &tmp_res);
if (!s.ok()) {
return s;
}
}
*res = member_result.size();
return Status::OK();
}
bool Nemo::SIsMember(const std::string &key, const std::string &member) {
std::string val;
std::string set_key = EncodeSetKey(key, member);
Status s = set_db_->Get(rocksdb::ReadOptions(), set_key, &val);
return s.ok();
}
//Note: no lock
Status Nemo::SInter(const std::vector<std::string> &keys, std::vector<std::string>& members) {
int numkey = keys.size();
if (numkey <= 0) {
return Status::InvalidArgument("SInter invalid parameter, no keys");
}
SIterator *iter = SScan(keys[0], -1, true);
for (; iter->Valid(); iter->Next()) {
int i = 1;
std::string member = iter->member();
for (; i < numkey; i++) {
if (!SIsMember(keys[i], member)) {
break;
}
}
if (i >= numkey) {
members.push_back(member);
}
}
set_db_->ReleaseSnapshot(iter->read_options().snapshot);
delete iter;
return Status::OK();
}
Status Nemo::SInterStore(const std::string &destination, const std::vector<std::string> &keys, int64_t *res) {
int numkey = keys.size();
//MutexLock l(&mutex_set_);
if (numkey <= 0) {
return Status::Corruption("SInter invalid parameter, no keys");
}
// TODO: maybe a MultiRecordLock is a better way;
std::set<std::string> lock_keys(keys.begin(), keys.end());
lock_keys.insert(destination);
for (auto iter = lock_keys.begin(); iter != lock_keys.end(); iter++) {
mutex_set_record_.Lock(*iter);
//printf ("SInter lock key(%s)\n", iter->c_str());
}
std::map<std::string, int> member_result;
std::map<std::string, int>::iterator it;
SIterator *iter = SScan(keys[0], -1, true);
for (; iter->Valid(); iter->Next()) {
int i = 1;
std::string member = iter->member();
for (; i < numkey; i++) {
if (!SIsMember(keys[i], member)) {
break;
}
}
if (i >= numkey) {
member_result[member] = 1;
}
}
set_db_->ReleaseSnapshot(iter->read_options().snapshot);
delete iter;
// we delete the destination if it exists
Status s;
int64_t tmp_res;
//RecordLock l(&mutex_set_record_, destination);
if (SCard(destination) > 0) {
SIterator *iter = SScan(destination, -1, true);
for (; iter->Valid(); iter->Next()) {
s = SRemNoLock(destination, iter->member(), &tmp_res);
if (!s.ok()) {
break;
//delete iter;
//return s;
}
}
set_db_->ReleaseSnapshot(iter->read_options().snapshot);
delete iter;
}
if (s.ok()) {
for (it = member_result.begin(); it != member_result.end(); it++) {
s = SAddNoLock(destination, it->first, &tmp_res);
if (!s.ok()) {
break;
}
}
}
for (auto iter = lock_keys.begin(); iter != lock_keys.end(); iter++) {
mutex_set_record_.Unlock(*iter);
}
*res = member_result.size();
return s;
}
// TODO need lock
Status Nemo::SDiff(const std::vector<std::string> &keys, std::vector<std::string>& members) {
int numkey = keys.size();
if (numkey <= 0) {
return Status::Corruption("SDiff invalid parameter, no keys");
}
SIterator *iter = SScan(keys[0], -1, true);
for (; iter->Valid(); iter->Next()) {
int i = 1;
std::string member = iter->member();
for (; i < numkey; i++) {
if (SIsMember(keys[i], member)) {
break;
}
}
if (i >= numkey) {
members.push_back(member);
}
}
set_db_->ReleaseSnapshot(iter->read_options().snapshot);
delete iter;
return Status::OK();
}
Status Nemo::SDiffStore(const std::string &destination, const std::vector<std::string> &keys, int64_t *res) {
int numkey = keys.size();
//MutexLock l(&mutex_set_);
if (numkey <= 0) {
return Status::Corruption("SDiff invalid parameter, no keys");
}
std::map<std::string, int> member_result;
std::map<std::string, int>::iterator it;
SIterator *iter = SScan(keys[0], -1, true);
for (; iter->Valid(); iter->Next()) {
int i = 1;
std::string member = iter->member();
for (; i < numkey; i++) {
if (SIsMember(keys[i], member)) {
break;
}
}
if (i >= numkey) {
member_result[member] = 1;
}
}
set_db_->ReleaseSnapshot(iter->read_options().snapshot);
delete iter;
// we delete the destination if it exists
Status s;
int64_t tmp_res;
RecordLock l(&mutex_set_record_, destination);
if (SCard(destination) > 0) {
SIterator *iter = SScan(destination, -1, true);
for (; iter->Valid(); iter->Next()) {
s = SRemNoLock(destination, iter->member(), &tmp_res);
if (!s.ok()) {
delete iter;
return s;
}
}
set_db_->ReleaseSnapshot(iter->read_options().snapshot);
delete iter;
}
for (it = member_result.begin(); it != member_result.end(); it++) {
s = SAddNoLock(destination, it->first, &tmp_res);
if (!s.ok()) {
return s;
}
}
*res = member_result.size();
return Status::OK();
}
int64_t Nemo::AddAndGetSpopCount(const std::string &key) {
std::map<std::string, int64_t> &spop_counts_map = spop_counts_store_.map_;
std::list<std::string> &spop_counts_list = spop_counts_store_.list_;
std::map<std::string, int64_t>::iterator iter;
int64_t spop_count;
MutexLock lm(&mutex_spop_counts_);
iter = spop_counts_map.find(key);
if (iter != spop_counts_map.end()) {
spop_count = ++iter->second;
} else {
spop_count = ++spop_counts_map[key];
spop_counts_list.push_back(key);
if (spop_counts_store_.cur_size_ >= spop_counts_store_.max_size_) {
spop_counts_map.erase(spop_counts_list.front());
spop_counts_list.erase(spop_counts_list.begin());
} else {
++spop_counts_store_.cur_size_;
}
}
return spop_count;
}
static inline int64_t NowMicros() {
struct timeval tv;
gettimeofday(&tv, NULL);
return static_cast<uint64_t>(tv.tv_sec) * 1000000 + tv.tv_usec;
}
void Nemo::ResetSpopCount(const std::string &key) {
MutexLock lm(&mutex_spop_counts_);
std::map<std::string, int64_t>::iterator iter = spop_counts_store_.map_.find(key);
if (iter == spop_counts_store_.map_.end()) {
return;
}
iter->second = 0;
return;
}
Status Nemo::SPop(const std::string &key, std::string &member) {
#define SPOP_COMPACT_THRESHOLD_COUNT 500
int card = SCard(key);
if (card <= 0) {
return Status::NotFound();
}
//MutexLock l(&mutex_set_);
uint64_t start_us = NowMicros(), duration_us;
srand (start_us);
int k = rand() % (card < 100 ? card : 100 ) + 1;
RecordLock l(&mutex_set_record_, key);
SIterator *iter = SScan(key, -1, true);
for (int i = 0; i < k - 1; i++) {
iter->Next();
}
member = iter->member();
set_db_->ReleaseSnapshot(iter->read_options().snapshot);
delete iter;
duration_us = NowMicros() - start_us;
int64_t spop_count = AddAndGetSpopCount(key);
if (spop_count >= SPOP_COMPACT_THRESHOLD_COUNT) {
AddBGTask({DBType::kSET_DB, OPERATION::kDEL_KEY, key, ""/*not used*/});
ResetSpopCount(key);
} else if (duration_us > 1000000UL) {
AddBGTask({DBType::kSET_DB, OPERATION::kDEL_KEY, key, ""/*not used*/});
}
int64_t res;
return SRemNoLock(key, member, &res);
}
//Note: no lock
Status Nemo::SRandMember(const std::string &key, std::vector<std::string> &members, const int count) {
if (key.size() >= KEY_MAX_LENGTH || key.size() <= 0) {
return Status::InvalidArgument("Invalid key length");
}
members.clear();
if (count == 0) {
return Status::OK();
}
int card = SCard(key);
if (card <= 0) {
return Status::NotFound();
}
std::map<int, int> idx_flag;
bool repeat_flag = false;
int remain_card = card;
int ncount = count;
if (ncount < 0) {
repeat_flag = true;
ncount = -ncount;
}
srand (time(NULL));
for (int ci = 0; ci < ncount; ci++) {
if (repeat_flag) {
int k = rand() % card;
idx_flag[k]++;
} else {
if (remain_card <= 0) break;
// everytime, we get the k-th remain idx
int k = rand() % remain_card + 1;
int i = 0;
int cnt = 0; // the valid k-th number
while (i < card && cnt < k) {
if (idx_flag.find(i) == idx_flag.end()) {
cnt++;
}
if (cnt == k) break;
i++;
}
idx_flag[i]++;
remain_card--;
}
}
SIterator *iter = SScan(key, -1, true);
for (int i = 0, cnt = 0; iter->Valid() && cnt < ncount; iter->Next(), i++) {
if (idx_flag.find(i) != idx_flag.end()) {
for (int j = 0; j < idx_flag[i]; j++) {
members.push_back(iter->member());
cnt++;
}
}
}
set_db_->ReleaseSnapshot(iter->read_options().snapshot);
delete iter;
return Status::OK();
}
Status Nemo::SMove(const std::string &source, const std::string &destination, const std::string &member, int64_t *res) {
if (source.size() >= KEY_MAX_LENGTH || source.size() <= 0) {
return Status::InvalidArgument("Invalid key length");
}
Status s;
if (source == destination) {
*res = 1;
return Status::OK();
}
//MutexLock l(&mutex_set_);
rocksdb::WriteBatch writebatch_s;
rocksdb::WriteBatch writebatch_d;
std::string source_key = EncodeSetKey(source, member);
std::string destination_key = EncodeSetKey(destination, member);
RecordLock l1(&mutex_set_record_, source);
RecordLock l2(&mutex_set_record_, destination);
std::string val;
s = set_db_->Get(rocksdb::ReadOptions(), source_key, &val);
if (s.ok()) {
*res = 1;
if (source != destination) {
if (IncrSSize(source, -1, writebatch_s) < 0) {
return Status::Corruption("incrSSize error");
}
writebatch_s.Delete(source_key);
s = set_db_->Get(rocksdb::ReadOptions(), destination_key, &val);
if (s.IsNotFound()) {
if (IncrSSize(destination, 1, writebatch_d) < 0) {
return Status::Corruption("incrSSize error");
}
}
writebatch_d.Put(destination_key, rocksdb::Slice());
s = set_db_->WriteWithOldKeyTTL(rocksdb::WriteOptions(), &(writebatch_s));
if (!s.ok()) {
return s;
}
s = set_db_->WriteWithOldKeyTTL(rocksdb::WriteOptions(), &(writebatch_d));
}
} else if (s.IsNotFound()) {
*res = 0;
} else {
return Status::Corruption("srem check member error");
}
return s;
}
Status Nemo::SDelKey(const std::string &key, int64_t *res) {
if (key.size() >= KEY_MAX_LENGTH || key.size() <= 0) {
return Status::InvalidArgument("Invalid key length");
}
Status s;
std::string val;
*res = 0;
std::string size_key = EncodeSSizeKey(key);
s = set_db_->Get(rocksdb::ReadOptions(), size_key, &val);
if (s.ok()) {
int64_t len = *(int64_t *)val.data();
if (len <= 0) {
s = Status::NotFound("");
} else {
len = 0;
*res = 1;
//MutexLock l(&mutex_set_);
s = set_db_->PutWithKeyVersion(rocksdb::WriteOptions(), size_key, rocksdb::Slice((char *)&len, sizeof(int64_t)));
}
}
return s;
}
Status Nemo::SExpire(const std::string &key, const int32_t seconds, int64_t *res) {
if (key.size() >= KEY_MAX_LENGTH || key.size() <= 0) {
return Status::InvalidArgument("Invalid key length");
}
Status s;
std::string val;
RecordLock l(&mutex_set_record_, key);
std::string size_key = EncodeSSizeKey(key);
s = set_db_->Get(rocksdb::ReadOptions(), size_key, &val);
if (s.IsNotFound()) {
*res = 0;
} else if (s.ok()) {
int64_t len = *(int64_t *)val.data();
if (len <= 0) {
return Status::NotFound("");
}
if (seconds > 0) {
//MutexLock l(&mutex_set_);
s = set_db_->Put(rocksdb::WriteOptions(), size_key, val, seconds);
} else {
int64_t count;
s = SDelKey(key, &count);
}
*res = 1;
}
return s;
}
Status Nemo::STTL(const std::string &key, int64_t *res) {
if (key.size() >= KEY_MAX_LENGTH || key.size() <= 0) {
return Status::InvalidArgument("Invalid key length");
}
Status s;
std::string val;
RecordLock l(&mutex_set_record_, key);
std::string size_key = EncodeSSizeKey(key);
s = set_db_->Get(rocksdb::ReadOptions(), size_key, &val);
if (s.IsNotFound()) {
*res = -2;
} else if (s.ok()) {
if (val.size() != sizeof(uint64_t) || *(int64_t *)val.data() <= 0) {
*res = -2;
return Status::NotFound("not found key");
}
int32_t ttl;
s = set_db_->GetKeyTTL(rocksdb::ReadOptions(), size_key, &ttl);
*res = ttl;
}
return s;
}
Status Nemo::SPersist(const std::string &key, int64_t *res) {
if (key.size() >= KEY_MAX_LENGTH || key.size() <= 0) {
return Status::InvalidArgument("Invalid key length");
}
Status s;
std::string val;
RecordLock l(&mutex_set_record_, key);
*res = 0;
std::string size_key = EncodeSSizeKey(key);
s = set_db_->Get(rocksdb::ReadOptions(), size_key, &val);
if (s.ok()) {
if (val.size() != sizeof(uint64_t) || *(int64_t *)val.data() <= 0) {
return Status::NotFound("not found key");
}
int32_t ttl;
s = set_db_->GetKeyTTL(rocksdb::ReadOptions(), size_key, &ttl);
if (s.ok() && ttl >= 0) {
//MutexLock l(&mutex_set_);
s = set_db_->Put(rocksdb::WriteOptions(), size_key, val);
*res = 1;
}
}
return s;
}
Status Nemo::SExpireat(const std::string &key, const int32_t timestamp, int64_t *res) {
if (key.size() >= KEY_MAX_LENGTH || key.size() <= 0) {
return Status::InvalidArgument("Invalid key length");
}
Status s;
std::string val;
RecordLock l(&mutex_set_record_, key);
std::string size_key = EncodeSSizeKey(key);
s = set_db_->Get(rocksdb::ReadOptions(), size_key, &val);
if (s.IsNotFound()) {
*res = 0;
} else if (s.ok()) {
int64_t len = *(int64_t *)val.data();
if (len <= 0) {
return Status::NotFound("");
}
std::time_t cur = std::time(0);
if (timestamp <= cur) {
int64_t count;
s = SDelKey(key, &count);
} else {
//MutexLock l(&mutex_set_);
s = set_db_->PutWithExpiredTime(rocksdb::WriteOptions(), size_key, val, timestamp);
}
*res = 1;
}
return s;
}
| {
"pile_set_name": "Github"
} |
// =============================================================================
// Scilab ( http://www.scilab.org/ ) - This file is part of Scilab
// Copyright (C) 2015 - Scilab Enterprises - Paul Bignier
// Copyright (C) ????-2008 - INRIA
//
// This file is distributed under the same license as the Scilab package.
// =============================================================================
//
// <-- CLI SHELL MODE -->
refMsg = msprintf(_("%s: Wrong number of input argument(s): %d expected.\n"), "scicosim", 6);
assert_checkerror("scicosim()", refMsg);
| {
"pile_set_name": "Github"
} |
sprite = lightsaberredtelek.png
blender = add
col_layer = -1
alpha = 128
on death()
remove()
on timer(1)
remove()
on detect_range(1, 0)
damage ( 0.035 )
| {
"pile_set_name": "Github"
} |
/*
* Copyright (c) 2012, dooApp <contact@dooapp.com>
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met:
*
* Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution.
* Neither the name of dooApp nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.dooapp.fxform;
import com.dooapp.fxform.annotation.NonVisual;
import javafx.beans.property.*;
import org.junit.Ignore;
/**
* Model object used for unit tests. Be careful when updating this bean, since most tests are based on its structure.
* <p/>
* User: Antoine Mischler <antoine@dooapp.com>
* Date: 12/09/11
* Time: 16:19
*/
@Ignore
public class TestBean {
private final StringProperty stringProperty = new SimpleStringProperty();
private final BooleanProperty booleanProperty = new SimpleBooleanProperty();
@NonVisual
private final IntegerProperty integerProperty = new SimpleIntegerProperty();
private final DoubleProperty doubleProperty = new SimpleDoubleProperty();
private final ObjectProperty<TestEnum> objectProperty = new SimpleObjectProperty<TestEnum>();
}
| {
"pile_set_name": "Github"
} |
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.spark.network.sasl;
import io.netty.channel.Channel;
import org.apache.spark.network.server.RpcHandler;
import org.apache.spark.network.server.TransportServerBootstrap;
import org.apache.spark.network.util.TransportConf;
/**
* A bootstrap which is executed on a TransportServer's client channel once a client connects
* to the server. This allows customizing the client channel to allow for things such as SASL
* authentication.
*/
public class SaslServerBootstrap implements TransportServerBootstrap {
private final TransportConf conf;
private final SecretKeyHolder secretKeyHolder;
public SaslServerBootstrap(TransportConf conf, SecretKeyHolder secretKeyHolder) {
this.conf = conf;
this.secretKeyHolder = secretKeyHolder;
}
/**
* Wrap the given application handler in a SaslRpcHandler that will handle the initial SASL
* negotiation.
*/
public RpcHandler doBootstrap(Channel channel, RpcHandler rpcHandler) {
return new SaslRpcHandler(conf, channel, rpcHandler, secretKeyHolder);
}
}
| {
"pile_set_name": "Github"
} |
Manifest-Version: 1.0
Bundle-ActivationPolicy: lazy
Bundle-ClassPath: .
Bundle-ManifestVersion: 2
Bundle-Name: Eclipse SmartHome SerialButton Binding
Bundle-RequiredExecutionEnvironment: JavaSE-1.8
Bundle-SymbolicName:
org.eclipse.smarthome.binding.serialbutton;singleton:=true
Bundle-Vendor: Eclipse.org/SmartHome
Bundle-Version: 0.11.0.qualifier
Export-Package:
org.eclipse.smarthome.binding.serialbutton,
org.eclipse.smarthome.binding.serialbutton.handler
Import-Package:
org.apache.commons.io,
org.eclipse.jdt.annotation;resolution:=optional,
org.eclipse.smarthome.binding.serialbutton,
org.eclipse.smarthome.binding.serialbutton.handler,
org.eclipse.smarthome.config.core,
org.eclipse.smarthome.core.library.types,
org.eclipse.smarthome.core.thing,
org.eclipse.smarthome.core.thing.binding,
org.eclipse.smarthome.core.thing.binding.builder,
org.eclipse.smarthome.core.thing.type,
org.eclipse.smarthome.core.types,
org.eclipse.smarthome.io.transport.serial,
org.slf4j
Service-Component: OSGI-INF/*.xml
Automatic-Module-Name: org.eclipse.smarthome.binding.serialbutton
| {
"pile_set_name": "Github"
} |
TARGET=$(shell ls *.py | grep -v test | grep -v parsetab.py)
ARGS=
PYTHON=python3
#PYTHON=python
#OPT=-m pdb
#OPT=-m cProfile -s time
#OPT=-m cProfile -o profile.rslt
.PHONY: all
all: test
.PHONY: run
run:
$(PYTHON) $(OPT) $(TARGET) $(ARGS)
.PHONY: test
test:
$(PYTHON) -m pytest -vv
.PHONY: check
check:
$(PYTHON) $(OPT) $(TARGET) $(ARGS) > tmp.v
iverilog -tnull -Wall tmp.v
rm -f tmp.v
.PHONY: clean
clean:
rm -rf *.pyc __pycache__ parsetab.py .cache *.out *.png *.dot tmp.v uut.vcd
| {
"pile_set_name": "Github"
} |
/*
Copyright 2015, Google Inc.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package orp.demo.urchat;
import android.util.Log;
import java.io.ByteArrayOutputStream;
import java.nio.ByteBuffer;
import orp.orp.Endpoint;
import orp.orp.Outbound;
import orp.orp.Session;
import orp.orp.SessionManager;
import orp.orp.TIDL;
/**
*/
public class UrChat {
public static enum State {
AWAITING_LOGIN,
AWAITING_COMMANDS
}
private static final short CHAT_PUBKEY_LEN = 70;
public static final short SEEKS_SIZE = 4;
public static final short SEEK_SIZE = 32;
public static final short SESSION_BUFFER_SIZE = 512;
public static final byte[] chat_hash =
{0x63, 0x68, 0x61, 0x74, 0xa, 0x0, 0x0, 0x0,
0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0,
0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0,
0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0};
public static final short chat_epnum = 0x1337;
private SessionManager sessionManager;
private Session session;
private State state;
private byte[] pubkey;
private UrChat() {
sessionManager = null;
session = null;
state = State.AWAITING_LOGIN;
pubkey = null;
}
public static UrChat getUrChat(SessionManager in_sessionManager) {
UrChat ret = new UrChat();
try {
ret.sessionManager = in_sessionManager;
Endpoint chat_ep = new Endpoint(chat_hash, (short) chat_epnum);
SessionManager.PreSession preSession = ret.sessionManager.newSession(chat_ep);
ret.session = preSession.getSession();
ret.state = State.AWAITING_LOGIN;
} catch (Exception e) {
e.printStackTrace();
return null;
}
return ret;
}
public byte[] getPubkey() {
if (State.AWAITING_COMMANDS != state) return null;
return pubkey;
}
public synchronized boolean login(byte[] pass) throws InterruptedException {
if (State.AWAITING_LOGIN != state) {
Log.d("UrChat", String.format("login failed: State.AWAITING_LOGIN != %s", state.toString()));
return false;
}
if (pass.length != 32) {
Log.d("UrChat", String.format("login failed: password length == %d != 32", pass.length));
return false;
}
try {
if (Outbound.State.SENT_ACK != session.writeBlocking(pass))
return false;
ByteBuffer response = ByteBuffer.wrap(session.read());
ChatResponse response_type = ChatResponse.Deserialize(response);
if (ChatResponse.CR_PUBKEY != response_type) return false;
short keylen = TIDL.uint16_deserialize(response);
if (CHAT_PUBKEY_LEN != keylen) return false;
pubkey = new byte[keylen];
for (int i = 0; i < pubkey.length; i++)
pubkey[i] = TIDL.uint8_deserialize(response);
} catch (Outbound.ETooMuchData eTooMuchData) {
eTooMuchData.printStackTrace();
return false;
} catch (TIDL.TIDLException e) {
e.printStackTrace();
return false;
}
Log.d("UrChat", "Logged in");
state = State.AWAITING_COMMANDS;
return true;
}
public synchronized boolean logout() throws InterruptedException {
try {
ByteArrayOutputStream command = new ByteArrayOutputStream();
ChatCommand.Serialize(command, ChatCommand.CC_LOGOUT);
if (Outbound.State.SENT_ACK != session.writeBlocking(command.toByteArray()))
return false;
ByteBuffer response = ByteBuffer.wrap(session.read());
ChatResponse response_type = ChatResponse.Deserialize(response);
if (ChatResponse.CR_GOODBYE != response_type) return false;
} catch (Outbound.ETooMuchData eTooMuchData) {
eTooMuchData.printStackTrace();
return false;
} catch (TIDL.TIDLException e) {
e.printStackTrace();
return false;
}
state = State.AWAITING_LOGIN;
pubkey = null;
Log.d("UrChat", "Logged out");
return true;
}
public synchronized boolean setSeek(byte[][] seeking) throws InterruptedException {
if (State.AWAITING_COMMANDS != state) return false;
if (!(seeking.length < SEEKS_SIZE)) {
Log.d("UrChat", String.format("setSeek failed: seeking.length == %d > %d == SEEKS_SIZE", seeking.length, SEEKS_SIZE));
return false;
}
for (int i = 0; i < seeking.length; i++) {
if (SEEK_SIZE != seeking[i].length) {
Log.d("UrChat", String.format("setSeek failed: seeking[i].length == %d != $d == SEEK_SIZE", seeking[i].length, SEEK_SIZE));
return false;
}
}
try {
ByteArrayOutputStream command = new ByteArrayOutputStream();
ChatCommand.Serialize(command, ChatCommand.CC_SETSEEK);
TIDL.uint32_serialize(command, seeking.length);
for (int i = 0; i < seeking.length; i++)
for (int j = 0; j < seeking[i].length; j++)
TIDL.uint8_serialize(command, seeking[i][j]);
if (Outbound.State.SENT_ACK != session.writeBlocking(command.toByteArray()))
return false;
ByteBuffer response = ByteBuffer.wrap(session.read());
ChatResponse response_type = ChatResponse.Deserialize(response);
if (ChatResponse.CR_SEEKUPDATED != response_type) return false;
} catch (Outbound.ETooMuchData eTooMuchData) {
eTooMuchData.printStackTrace();
return false;
} catch (TIDL.TIDLException e) {
e.printStackTrace();
return false;
}
Log.d("UrChat", String.format("Set seek (%d active)", seeking.length));
return true;
}
public synchronized byte[] getSeek(int seeknum) throws InterruptedException {
try {
ByteArrayOutputStream command = new ByteArrayOutputStream();
ChatCommand.Serialize(command, ChatCommand.CC_GETSEEK);
TIDL.uint32_serialize(command, seeknum);
if (Outbound.State.SENT_ACK != session.writeBlocking(command.toByteArray()))
return null;
ByteBuffer response = ByteBuffer.wrap(session.read());
ChatResponse response_type = ChatResponse.Deserialize(response);
if (ChatResponse.CR_SEEK != response_type) return null;
byte[] ret = new byte[SESSION_BUFFER_SIZE];
for (int i = 0; i < SESSION_BUFFER_SIZE; i++)
ret[i] = TIDL.uint8_deserialize(response);
Log.d("UrChat", String.format("Fetched seek string for seek-slot %d", seeknum));
return ret;
} catch (Outbound.ETooMuchData eTooMuchData) {
eTooMuchData.printStackTrace();
} catch (TIDL.TIDLException e) {
e.printStackTrace();
}
return null;
}
public static final int INVALID_CHANNEL = -1;
public synchronized int getChannel(byte[] remote_pubkey) throws InterruptedException {
if (CHAT_PUBKEY_LEN != remote_pubkey.length) return INVALID_CHANNEL;
try {
ByteArrayOutputStream command = new ByteArrayOutputStream();
ChatCommand.Serialize(command, ChatCommand.CC_GET_CHANNEL);
TIDL.uint16_serialize(command, (short) remote_pubkey.length);
for (int i = 0; i < remote_pubkey.length; i++)
TIDL.uint8_serialize(command, remote_pubkey[i]);
if (Outbound.State.SENT_ACK != session.writeBlocking(command.toByteArray()))
return INVALID_CHANNEL;
ByteBuffer response = ByteBuffer.wrap(session.read());
ChatResponse response_type = ChatResponse.Deserialize(response);
if (ChatResponse.CR_CHANNEL != response_type) return INVALID_CHANNEL;
int chan = TIDL.uint32_deserialize(response);
Log.d("UrChat", String.format("Obtained channel (%d) with contact", chan));
return chan;
} catch (Outbound.ETooMuchData eTooMuchData) {
eTooMuchData.printStackTrace();
} catch (TIDL.TIDLException e) {
e.printStackTrace();
}
return INVALID_CHANNEL;
}
public synchronized boolean closeChannel(int channel_id) throws InterruptedException {
try {
ByteArrayOutputStream command = new ByteArrayOutputStream();
ChatCommand.Serialize(command, ChatCommand.CC_CLOSE_CHANNEL);
TIDL.uint32_serialize(command, channel_id);
if (Outbound.State.SENT_ACK != session.writeBlocking(command.toByteArray()))
return false;
ByteBuffer response = ByteBuffer.wrap(session.read());
ChatResponse response_type = ChatResponse.Deserialize(response);
if (ChatResponse.CR_CHANNEL_CLOSED != response_type) return false;
} catch (Outbound.ETooMuchData eTooMuchData) {
eTooMuchData.printStackTrace();
return false;
} catch (TIDL.TIDLException e) {
e.printStackTrace();
return false;
}
Log.d("UrChat", String.format("Closed channel %d", channel_id));
return true;
}
public synchronized byte[] getExchange(int channel_id) throws InterruptedException {
try {
ByteArrayOutputStream command = new ByteArrayOutputStream();
ChatCommand.Serialize(command, ChatCommand.CC_GET_EXCHANGE);
TIDL.uint32_serialize(command, channel_id);
if (Outbound.State.SENT_ACK != session.writeBlocking(command.toByteArray()))
return null;
ByteBuffer response = ByteBuffer.wrap(session.read());
ChatResponse response_type = ChatResponse.Deserialize(response);
if (ChatResponse.CR_EXCHANGE != response_type) return null;
byte[] ret = new byte[SESSION_BUFFER_SIZE];
for (int i = 0; i < SESSION_BUFFER_SIZE; i++)
ret[i] = TIDL.uint8_deserialize(response);
Log.d("UrChat", String.format("Obtained exchange string for channel ", channel_id));
return ret;
} catch (Outbound.ETooMuchData eTooMuchData) {
eTooMuchData.printStackTrace();
} catch (TIDL.TIDLException e) {
e.printStackTrace();
}
return null;
}
public synchronized byte[] encrypt(int channel_id, String message) throws InterruptedException {
try {
ByteArrayOutputStream command = new ByteArrayOutputStream();
ChatCommand.Serialize(command, ChatCommand.CC_ENCRYPT);
TIDL.uint32_serialize(command, channel_id);
TIDL.string_serialize(command, message);
if (Outbound.State.SENT_ACK != session.writeBlocking(command.toByteArray()))
return null;
ByteBuffer response = ByteBuffer.wrap(session.read());
ChatResponse response_type = ChatResponse.Deserialize(response);
if (ChatResponse.CR_CIPHERTEXT != response_type) return null;
byte[] ret = new byte[SESSION_BUFFER_SIZE];
for (int i = 0; i < SESSION_BUFFER_SIZE; i++)
ret[i] = TIDL.uint8_deserialize(response);
Log.d("UrChat", String.format("Encrypted message for channel %d", channel_id));
return ret;
} catch (Outbound.ETooMuchData eTooMuchData) {
eTooMuchData.printStackTrace();
} catch (TIDL.TIDLException e) {
e.printStackTrace();
}
return null;
}
public static class Decrypted {
public static enum Kind {
SEEK_FOUND,
EXCHANGE_OCCURRED,
PLAINTEXT_GOT,
NEVERMIND
}
public final Kind kind;
public final int seek;
public final byte[] pubkey;
public final int channel_id;
public final String message;
private Decrypted(int in_seek, byte[] in_pubkey) {
kind = Kind.SEEK_FOUND;
seek = in_seek;
pubkey = in_pubkey;
channel_id = INVALID_CHANNEL;
message = null;
}
private Decrypted(int in_channel_id) {
kind = Kind.EXCHANGE_OCCURRED;
seek = -1;
pubkey = null;
channel_id = in_channel_id;
message = null;
}
private Decrypted(int in_channel_id, String in_message) {
kind = Kind.PLAINTEXT_GOT;
seek = -1;
pubkey = null;
channel_id = in_channel_id;
message = in_message;
}
private Decrypted() {
kind = Kind.NEVERMIND;
seek = -1;
pubkey = null;
channel_id = INVALID_CHANNEL;
message = null;
}
protected static Decrypted seekFound(int in_seek, byte[] in_pubkey) {
return new Decrypted(in_seek, in_pubkey);
}
protected static Decrypted exchangeOccurred(int in_channel_id) {
return new Decrypted(in_channel_id);
}
protected static Decrypted plaintextGot(int in_channel_id, String in_message) {
return new Decrypted(in_channel_id, in_message);
}
protected static Decrypted nevermind() {
return new Decrypted();
}
}
public synchronized Decrypted incoming(byte[] ciphertext) throws InterruptedException {
if (SESSION_BUFFER_SIZE != ciphertext.length) return null;
try {
ByteArrayOutputStream command = new ByteArrayOutputStream();
ChatCommand.Serialize(command, ChatCommand.CC_INCOMING);
for (int i = 0; i < ciphertext.length; i++)
TIDL.uint8_serialize(command, ciphertext[i]);
if (Outbound.State.SENT_ACK != session.writeBlocking(command.toByteArray()))
return null;
ByteBuffer response = ByteBuffer.wrap(session.read());
ChatResponse response_type = ChatResponse.Deserialize(response);
switch (response_type) {
case CR_FOUND: {
int seeknum = TIDL.uint32_deserialize(response);
short keylen = TIDL.uint16_deserialize(response);
if (CHAT_PUBKEY_LEN != keylen) return null;
byte[] pubkey = new byte[keylen];
for (int i = 0; i < pubkey.length; i++)
pubkey[i] = TIDL.uint8_deserialize(response);
Log.d("UrChat", String.format("Seek found (seeknum %d)", seeknum));
return Decrypted.seekFound(seeknum, pubkey);
}
case CR_EXCHANGED: {
int channel_id = TIDL.uint32_deserialize(response);
Log.d("UrChat", String.format("Exchange occurred (channel %d)", channel_id));
return Decrypted.exchangeOccurred(channel_id);
}
case CR_PLAINTEXT: {
int channel_id = TIDL.uint32_deserialize(response);
String message = TIDL.string_deserialize(response);
Log.d("UrChat", String.format("Plaintext got (channel %d)", channel_id));
return Decrypted.plaintextGot(channel_id, message);
}
case CR_NEVERMIND:
return Decrypted.nevermind();
default:
return null;
}
} catch (Outbound.ETooMuchData eTooMuchData) {
eTooMuchData.printStackTrace();
} catch (TIDL.TIDLException e) {
e.printStackTrace();
}
return null;
}
}
| {
"pile_set_name": "Github"
} |
distributionUrl=https://repo1.maven.org/maven2/org/apache/maven/apache-maven/3.5.0/apache-maven-3.5.0-bin.zip
| {
"pile_set_name": "Github"
} |
#ifndef POT_H
#define POT_H
#include "ConnectedComponent.h"
class Pot:public ConnectedComponent
{
public:
Pot(int,int,Component *);
~Pot();
Pin * PortSelected ();
void Select (bool);
void Paint(HDC _hdc, PAINTSTRUCT _ps, HDC _hdcMemory);
void HandleMenu ( int command );
void HandleMouseMove (HWND hWnd, int _x, int _y);
void HandleMouseDown (HWND hWnd, int _x, int _y);
void HandleMouseUp (HWND hWnd);
void Reset();
bool IsSet();
void MoveTo (int, int);
void Init (HWND _windowHandle, HINSTANCE _g_hInst, char *);
Pin * PinActive ();
Pin * gnd;
Pin * power;
Pin * analogInput;
void AddMenu ();
void SaveYourself (FILE * fp);
Pin * FindPort ( char *);
void CleanUp();
private:
bool offOn;
};
#endif
| {
"pile_set_name": "Github"
} |
# Copyright 2012 Mozilla Foundation
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# Main toolbar buttons (tooltips and alt text for images)
previous.title=Pot buk mukato
previous_label=Mukato
next.title=Pot buk malubo
next_label=Malubo
# LOCALIZATION NOTE (page_label, page_of):
# These strings are concatenated to form the "Page: X of Y" string.
# Do not translate "{{pageCount}}", it will be substituted with a number
# representing the total number of pages.
page_label=Pot buk:
page_of=pi {{pageCount}}
zoom_out.title=Jwik Matidi
zoom_out_label=Jwik Matidi
zoom_in.title=Kwot Madit
zoom_in_label=Kwot Madit
zoom.title=Kwoti
presentation_mode.title=Lokke i kit me tyer
presentation_mode_label=Kit me tyer
open_file.title=Yab Pwail
open_file_label=Yab
print.title=Go
print_label=Go
download.title=Gam
download_label=Gam
bookmark.title=Neno ma kombedi (lok onyo yab i dirica manyen)
bookmark_label=Neno ma kombedi
# Secondary toolbar and context menu
tools.title=Gintic
tools_label=Gintic
first_page.title=Cit i pot buk mukwongo
first_page.label=Cit i pot buk mukwongo
first_page_label=Cit i pot buk mukwongo
last_page.title=Cit i pot buk magiko
last_page.label=Cit i pot buk magiko
last_page_label=Cit i pot buk magiko
page_rotate_cw.title=Wire i tung lacuc
page_rotate_cw.label=Wire i tung lacuc
page_rotate_cw_label=Wire i tung lacuc
page_rotate_ccw.title=Wire i tung lacam
page_rotate_ccw.label=Wire i tung lacam
page_rotate_ccw_label=Wire i tung lacam
hand_tool_enable.title=Ye gintic me cing
hand_tool_enable_label=Ye gintic me cing
hand_tool_disable.title=Juk gintic me cing
hand_tool_disable_label=Juk gintic me cing
# Document properties dialog box
document_properties.title=Jami me gin acoya…
document_properties_label=Jami me gin acoya…
document_properties_file_name=Nying pwail:
document_properties_file_size=Dit pa pwail:
document_properties_kb={{size_kb}} KB ({{size_b}} bytes)
document_properties_mb={{size_mb}} MB ({{size_b}} bytes)
document_properties_title=Wiye:
document_properties_author=Ngat mucoyo:
document_properties_subject=Lok:
document_properties_keywords=Lok mapire tek:
document_properties_creation_date=Nino dwe me cwec:
document_properties_modification_date=Nino dwe me yub:
document_properties_date_string={{date}}, {{time}}
document_properties_creator=Lacwec:
document_properties_producer=Layub PDF:
document_properties_version=Kit PDF:
document_properties_page_count=Kwan me pot buk:
document_properties_close=Lor
# Tooltips and alt text for side panel toolbar buttons
# (the _label strings are alt text for the buttons, the .title strings are
# tooltips)
toggle_sidebar.title=Lok gintic ma inget
toggle_sidebar_label=Lok gintic ma inget
outline.title=Nyut rek pa gin acoya
outline_label=Pek pa gin acoya
attachments.title=Nyut twec
attachments_label=Twec
thumbs.title=Nyut cal
thumbs_label=Cal
findbar.title=Nong iye gin acoya
findbar_label=Nong
# Thumbnails panel item (tooltip and alt text for images)
# LOCALIZATION NOTE (thumb_page_title): "{{page}}" will be replaced by the page
# number.
thumb_page_title=Pot buk {{page}}
# LOCALIZATION NOTE (thumb_page_canvas): "{{page}}" will be replaced by the page
# number.
thumb_page_canvas=Cal me pot buk {{page}}
# Find panel button title and messages
find_label=Nong:
find_previous.title=Nong timme pa lok mukato
find_previous_label=Mukato
find_next.title=Nong timme pa lok malubo
find_next_label=Malubo
find_highlight=Wer weng
find_match_case_label=Lok marwate
find_reached_top=Oo iwi gin acoya, omede ki i tere
find_reached_bottom=Oo i agiki me gin acoya, omede ki iwiye
find_not_found=Lok pe ononge
# Error panel labels
error_more_info=Ngec Mukene
error_less_info=Ngec Manok
error_close=Lor
# LOCALIZATION NOTE (error_version_info): "{{version}}" and "{{build}}" will be
# replaced by the PDF.JS version and build ID.
error_version_info=PDF.js v{{version}} (build: {{build}})
# LOCALIZATION NOTE (error_message): "{{message}}" will be replaced by an
# english string describing the error.
error_message=Kwena: {{message}}
# LOCALIZATION NOTE (error_stack): "{{stack}}" will be replaced with a stack
# trace.
error_stack=Can kikore {{stack}}
# LOCALIZATION NOTE (error_file): "{{file}}" will be replaced with a filename
error_file=Pwail: {{file}}
# LOCALIZATION NOTE (error_line): "{{line}}" will be replaced with a line number
error_line=Rek: {{line}}
rendering_error=Bal otime i kare me nyuto pot buk.
# Predefined zoom values
page_scale_width=Lac me iye pot buk
page_scale_fit=Porre me pot buk
page_scale_auto=Kwot pire kene
page_scale_actual=Dite kikome
# LOCALIZATION NOTE (page_scale_percent): "{{scale}}" will be replaced by a
# numerical scale value.
page_scale_percent={{scale}}%
# Loading indicator messages
loading_error_indicator=Bal
loading_error=Bal otime kun cano PDF.
invalid_file_error=Pwail me PDF ma pe atir onyo obale woko.
missing_file_error=Pwail me PDF tye ka rem.
unexpected_response_error=Lagam mape kigeno pa lapok tic
# LOCALIZATION NOTE (text_annotation_type.alt): This is used as a tooltip.
# "{{type}}" will be replaced with an annotation type from a list defined in
# the PDF spec (32000-1:2008 Table 169 – Annotation types).
# Some common types are e.g.: "Check", "Text", "Comment", "Note"
text_annotation_type.alt=[{{type}} Lok angea manok]
password_label=Ket mung me donyo me yabo pwail me PDF man.
password_invalid=Mung me donyo pe atir. Tim ber i tem doki.
password_ok=OK
password_cancel=Juk
printing_not_supported=Ciko: Layeny ma pe teno goyo liweng.
printing_not_ready=Ciko: PDF pe ocane weng me agoya.
web_fonts_disabled=Kijuko dit pa coc me kakube woko: pe romo tic ki dit pa coc me PDF ma kiketo i kine.
document_colors_disabled=Pe ki ye ki gin acoya me PDF me tic ki rangi gi kengi: 'Ye pot buk me yero rangi mamegi kengi' kijuko woko i layeny.
| {
"pile_set_name": "Github"
} |
<annotation>
<folder>imagesRaw</folder>
<filename>2017-12-25 23:31:53.900226.jpg</filename>
<path>/Users/abell/Development/other.nyc/Camera/imagesRaw/2017-12-25 23:31:53.900226.jpg</path>
<source>
<database>Unknown</database>
</source>
<size>
<width>352</width>
<height>240</height>
<depth>1</depth>
</size>
<segmented>0</segmented>
<object>
<name>bus</name>
<pose>Unspecified</pose>
<truncated>1</truncated>
<difficult>0</difficult>
<bndbox>
<xmin>1</xmin>
<ymin>153</ymin>
<xmax>97</xmax>
<ymax>240</ymax>
</bndbox>
</object>
<object>
<name>car</name>
<pose>Unspecified</pose>
<truncated>0</truncated>
<difficult>0</difficult>
<bndbox>
<xmin>201</xmin>
<ymin>145</ymin>
<xmax>220</xmax>
<ymax>161</ymax>
</bndbox>
</object>
</annotation>
| {
"pile_set_name": "Github"
} |
struct v2f {
vec4 pos;
vec2 uv;
vec4 color;
};
struct a2v {
vec4 pos;
vec2 uv;
vec3 normal;
vec4 color;
};
uniform mat4 mvp;
v2f xlat_main( in a2v v );
v2f xlat_main( in a2v v ) {
v2f o;
float dx;
float dy;
float dz;
o.pos = ( mvp * v.pos );
o.color = v.color;
o.color.xyz += v.normal;
o.uv = v.uv;
dx = v.pos.x ;
dy = v.pos.y ;
dz = v.pos.z ;
return o;
}
varying vec2 xlv_TEXCOORD0;
varying vec4 xlv_COLOR;
void main() {
v2f xl_retval;
a2v xlt_v;
xlt_v.pos = vec4( gl_Vertex);
xlt_v.uv = vec2( gl_MultiTexCoord0);
xlt_v.normal = vec3( gl_Normal);
xlt_v.color = vec4( gl_Color);
xl_retval = xlat_main( xlt_v);
gl_Position = vec4( xl_retval.pos);
xlv_TEXCOORD0 = vec2( xl_retval.uv);
xlv_COLOR = vec4( xl_retval.color);
}
| {
"pile_set_name": "Github"
} |
changelog:
- type: NON_USER_FACING
description: Add note about OPA version logging to docs
issueLink: https://github.com/solo-io/gloo/issues/3136
resolvesIssue: false
| {
"pile_set_name": "Github"
} |
package com.packt.patterninspring.chapter11.web.reactive.function;
import java.net.URI;
import java.util.List;
import org.springframework.http.HttpMethod;
import org.springframework.http.client.reactive.ReactorClientHttpConnector;
import org.springframework.web.reactive.function.BodyInserters;
import org.springframework.web.reactive.function.client.ClientRequest;
import org.springframework.web.reactive.function.client.ClientResponse;
import org.springframework.web.reactive.function.client.ExchangeFunction;
import org.springframework.web.reactive.function.client.ExchangeFunctions;
import com.packt.patterninspring.chapter11.web.reactive.model.Account;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
public class Client {
private ExchangeFunction exchange = ExchangeFunctions.create(new ReactorClientHttpConnector());
public static void main(String[] args) throws Exception {
Client client = new Client();
client.createAccount();
client.findAllAccounts();
}
public void findAllAccounts() {
URI uri = URI.create(String.format("http://%s:%d/account", Server.HOST, Server.TOMCAT_PORT));
ClientRequest request = ClientRequest.method(HttpMethod.GET, uri).build();
Flux<Account> account = exchange.exchange(request)
.flatMapMany(response -> response.bodyToFlux(Account.class));
Mono<List<Account>> accountList = account.collectList();
System.out.println(accountList.block());
}
public void createAccount() {
URI uri = URI.create(String.format("http://%s:%d/account", Server.HOST, Server.TOMCAT_PORT));
Account account = new Account(5000l, "Arnav Rajput", 500000l, "Sector-5");
ClientRequest request = ClientRequest.method(HttpMethod.POST, uri)
.body(BodyInserters.fromObject(account)).build();
Mono<ClientResponse> response = exchange.exchange(request);
System.out.println(response.block().statusCode());
}
}
| {
"pile_set_name": "Github"
} |
{
"Names": {
"BR": "Parahi",
"CN": "Haina",
"DE": "Tiamana",
"FR": "Wīwī",
"GB": "Hononga o Piritene",
"IN": "Inia",
"IT": "Itāria",
"JP": "Hapani",
"MK": "Makerōnia ki te Raki",
"NZ": "Aotearoa",
"RU": "Rūhia",
"US": "Hononga o Amerika"
}
}
| {
"pile_set_name": "Github"
} |
// Go support for leveled logs, analogous to https://code.google.com/p/google-glog/
//
// Copyright 2013 Google Inc. All Rights Reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
// File I/O for logs.
package klog
import (
"errors"
"fmt"
"os"
"os/user"
"path/filepath"
"strings"
"sync"
"time"
)
// MaxSize is the maximum size of a log file in bytes.
var MaxSize uint64 = 1024 * 1024 * 1800
// logDirs lists the candidate directories for new log files.
var logDirs []string
func createLogDirs() {
if logging.logDir != "" {
logDirs = append(logDirs, logging.logDir)
}
logDirs = append(logDirs, os.TempDir())
}
var (
pid = os.Getpid()
program = filepath.Base(os.Args[0])
host = "unknownhost"
userName = "unknownuser"
)
func init() {
h, err := os.Hostname()
if err == nil {
host = shortHostname(h)
}
current, err := user.Current()
if err == nil {
userName = current.Username
}
// Sanitize userName since it may contain filepath separators on Windows.
userName = strings.Replace(userName, `\`, "_", -1)
}
// shortHostname returns its argument, truncating at the first period.
// For instance, given "www.google.com" it returns "www".
func shortHostname(hostname string) string {
if i := strings.Index(hostname, "."); i >= 0 {
return hostname[:i]
}
return hostname
}
// logName returns a new log file name containing tag, with start time t, and
// the name for the symlink for tag.
func logName(tag string, t time.Time) (name, link string) {
name = fmt.Sprintf("%s.%s.%s.log.%s.%04d%02d%02d-%02d%02d%02d.%d",
program,
host,
userName,
tag,
t.Year(),
t.Month(),
t.Day(),
t.Hour(),
t.Minute(),
t.Second(),
pid)
return name, program + "." + tag
}
var onceLogDirs sync.Once
// create creates a new log file and returns the file and its filename, which
// contains tag ("INFO", "FATAL", etc.) and t. If the file is created
// successfully, create also attempts to update the symlink for that tag, ignoring
// errors.
// The startup argument indicates whether this is the initial startup of klog.
// If startup is true, existing files are opened for appending instead of truncated.
func create(tag string, t time.Time, startup bool) (f *os.File, filename string, err error) {
if logging.logFile != "" {
f, err := openOrCreate(logging.logFile, startup)
if err == nil {
return f, logging.logFile, nil
}
return nil, "", fmt.Errorf("log: unable to create log: %v", err)
}
onceLogDirs.Do(createLogDirs)
if len(logDirs) == 0 {
return nil, "", errors.New("log: no log dirs")
}
name, link := logName(tag, t)
var lastErr error
for _, dir := range logDirs {
fname := filepath.Join(dir, name)
f, err := openOrCreate(fname, startup)
if err == nil {
symlink := filepath.Join(dir, link)
os.Remove(symlink) // ignore err
os.Symlink(name, symlink) // ignore err
return f, fname, nil
}
lastErr = err
}
return nil, "", fmt.Errorf("log: cannot create log: %v", lastErr)
}
// The startup argument indicates whether this is the initial startup of klog.
// If startup is true, existing files are opened for appending instead of truncated.
func openOrCreate(name string, startup bool) (*os.File, error) {
if startup {
f, err := os.OpenFile(name, os.O_RDWR|os.O_CREATE|os.O_APPEND, 0666)
return f, err
}
f, err := os.Create(name)
return f, err
}
| {
"pile_set_name": "Github"
} |
Thin wrapper around `react-select` to apply our Opentrons-specific styles. All props are passed directly to [`react-select`](https://react-select.com/props) except for `styles`, `components`, and `classNamePrefix`. The `className` prop appends to the `className` we pass `react-select`. Those props are not passed directly because they provide the base styling of the component
```js
<Select
options={[
{ value: 'foo', label: 'Foo!' },
{ value: 'bar', label: 'Bar?', isDisabled: true },
{ value: 'baz', label: 'Baz?!' },
]}
/>
```
You can also pass grouped options:
```js
<Select
options={[
{
label: 'Classics',
options: [
{ value: 'foo', label: 'Foo!' },
{ value: 'bar', label: 'Bar?' },
],
},
{
label: 'Modern',
options: [
{ value: 'baz', label: 'Baz?!' },
{ value: 'quux', label: 'Quux.' },
],
},
]}
/>
```
Passing `value` controls the input. **Note that `value` has the same format as an entry in `options`**:
```js
<Select
value={{ value: 'bar', label: 'Bar?' }}
options={[
{ value: 'foo', label: 'Foo!' },
{ value: 'bar', label: 'Bar?' },
{ value: 'baz', label: 'Baz?!' },
]}
/>
```
You can control the renders of individual options with the `formatOptionLabel` prop:
```js
<Select
options={[
{ value: 'foo', label: 'Foo!' },
{ value: 'bar', label: 'Bar?' },
{ value: 'baz', label: 'Baz?!' },
]}
formatOptionLabel={(option, { context }) =>
context === 'menu' && option.value === 'bar' ? (
<span style={{ color: 'green' }}>{option.label}</span>
) : (
option.label
)
}
/>
```
To override any styling, we use [`react-select`'s BEM](https://react-select.com/styles#using-classnames) class names with our specific prefix, which is `ot_select`. See `SelectField` for a specific example, where the background color of the `Control` element is modified if the field has an error
```css
.my_class_name {
& :global(.ot_select__control) {
background-color: blue;
}
}
```
```js static
<Select
className={styles.my_class_name}
options={[
{ value: 'foo', label: 'Foo!' },
{ value: 'bar', label: 'Bar?' },
{ value: 'baz', label: 'Baz?!' },
]}
/>
```
| {
"pile_set_name": "Github"
} |
/*###ICF### Section handled by ICF editor, don't touch! ****/
/*-Editor annotation file-*/
/* IcfEditorFile="$TOOLKIT_DIR$\config\ide\IcfEditor\cortex_v1_0.xml" */
/*-Specials-*/
define symbol __ICFEDIT_intvec_start__ = 0x08100000;
/*-Memory Regions-*/
define symbol __ICFEDIT_region_ROM_start__ = 0x08100000;
define symbol __ICFEDIT_region_ROM_end__ = 0x081FFFFF;
define symbol __ICFEDIT_region_RAM_start__ = 0x10000000;
define symbol __ICFEDIT_region_RAM_end__ = 0x10047FFF;
/*-Sizes-*/
define symbol __ICFEDIT_size_cstack__ = 0x400;
define symbol __ICFEDIT_size_heap__ = 0x200;
/**** End of ICF editor section. ###ICF###*/
define memory mem with size = 4G;
define region ROM_region = mem:[from __ICFEDIT_region_ROM_start__ to __ICFEDIT_region_ROM_end__];
define region RAM_region = mem:[from __ICFEDIT_region_RAM_start__ to __ICFEDIT_region_RAM_end__];
define block CSTACK with alignment = 8, size = __ICFEDIT_size_cstack__ { };
define block HEAP with alignment = 8, size = __ICFEDIT_size_heap__ { };
initialize by copy { readwrite };
do not initialize { section .noinit };
place at address mem:__ICFEDIT_intvec_start__ { readonly section .intvec };
place in ROM_region { readonly };
place in RAM_region { readwrite,
block CSTACK, block HEAP }; | {
"pile_set_name": "Github"
} |
// mkerrors.sh -maix64
// Code generated by the command above; see README.md. DO NOT EDIT.
// +build ppc64,aix
// Code generated by cmd/cgo -godefs; DO NOT EDIT.
// cgo -godefs -- -maix64 _const.go
package unix
import "syscall"
const (
AF_APPLETALK = 0x10
AF_BYPASS = 0x19
AF_CCITT = 0xa
AF_CHAOS = 0x5
AF_DATAKIT = 0x9
AF_DECnet = 0xc
AF_DLI = 0xd
AF_ECMA = 0x8
AF_HYLINK = 0xf
AF_IMPLINK = 0x3
AF_INET = 0x2
AF_INET6 = 0x18
AF_INTF = 0x14
AF_ISO = 0x7
AF_LAT = 0xe
AF_LINK = 0x12
AF_LOCAL = 0x1
AF_MAX = 0x1e
AF_NDD = 0x17
AF_NETWARE = 0x16
AF_NS = 0x6
AF_OSI = 0x7
AF_PUP = 0x4
AF_RIF = 0x15
AF_ROUTE = 0x11
AF_SNA = 0xb
AF_UNIX = 0x1
AF_UNSPEC = 0x0
ALTWERASE = 0x400000
ARPHRD_802_3 = 0x6
ARPHRD_802_5 = 0x6
ARPHRD_ETHER = 0x1
ARPHRD_FDDI = 0x1
B0 = 0x0
B110 = 0x3
B1200 = 0x9
B134 = 0x4
B150 = 0x5
B1800 = 0xa
B19200 = 0xe
B200 = 0x6
B2400 = 0xb
B300 = 0x7
B38400 = 0xf
B4800 = 0xc
B50 = 0x1
B600 = 0x8
B75 = 0x2
B9600 = 0xd
BRKINT = 0x2
BS0 = 0x0
BS1 = 0x1000
BSDLY = 0x1000
CAP_AACCT = 0x6
CAP_ARM_APPLICATION = 0x5
CAP_BYPASS_RAC_VMM = 0x3
CAP_CLEAR = 0x0
CAP_CREDENTIALS = 0x7
CAP_EFFECTIVE = 0x1
CAP_EWLM_AGENT = 0x4
CAP_INHERITABLE = 0x2
CAP_MAXIMUM = 0x7
CAP_NUMA_ATTACH = 0x2
CAP_PERMITTED = 0x3
CAP_PROPAGATE = 0x1
CAP_PROPOGATE = 0x1
CAP_SET = 0x1
CBAUD = 0xf
CFLUSH = 0xf
CIBAUD = 0xf0000
CLOCAL = 0x800
CLOCK_MONOTONIC = 0xa
CLOCK_PROCESS_CPUTIME_ID = 0xb
CLOCK_REALTIME = 0x9
CLOCK_THREAD_CPUTIME_ID = 0xc
CR0 = 0x0
CR1 = 0x100
CR2 = 0x200
CR3 = 0x300
CRDLY = 0x300
CREAD = 0x80
CS5 = 0x0
CS6 = 0x10
CS7 = 0x20
CS8 = 0x30
CSIOCGIFCONF = -0x3fef96dc
CSIZE = 0x30
CSMAP_DIR = "/usr/lib/nls/csmap/"
CSTART = '\021'
CSTOP = '\023'
CSTOPB = 0x40
CSUSP = 0x1a
ECHO = 0x8
ECHOCTL = 0x20000
ECHOE = 0x10
ECHOK = 0x20
ECHOKE = 0x80000
ECHONL = 0x40
ECHOPRT = 0x40000
ECH_ICMPID = 0x2
ETHERNET_CSMACD = 0x6
EVENP = 0x80
EXCONTINUE = 0x0
EXDLOK = 0x3
EXIO = 0x2
EXPGIO = 0x0
EXRESUME = 0x2
EXRETURN = 0x1
EXSIG = 0x4
EXTA = 0xe
EXTB = 0xf
EXTRAP = 0x1
EYEC_RTENTRYA = 0x257274656e747241
EYEC_RTENTRYF = 0x257274656e747246
E_ACC = 0x0
FD_CLOEXEC = 0x1
FD_SETSIZE = 0xfffe
FF0 = 0x0
FF1 = 0x2000
FFDLY = 0x2000
FLUSHBAND = 0x40
FLUSHLOW = 0x8
FLUSHO = 0x100000
FLUSHR = 0x1
FLUSHRW = 0x3
FLUSHW = 0x2
F_CLOSEM = 0xa
F_DUP2FD = 0xe
F_DUPFD = 0x0
F_GETFD = 0x1
F_GETFL = 0x3
F_GETLK = 0xb
F_GETLK64 = 0xb
F_GETOWN = 0x8
F_LOCK = 0x1
F_OK = 0x0
F_RDLCK = 0x1
F_SETFD = 0x2
F_SETFL = 0x4
F_SETLK = 0xc
F_SETLK64 = 0xc
F_SETLKW = 0xd
F_SETLKW64 = 0xd
F_SETOWN = 0x9
F_TEST = 0x3
F_TLOCK = 0x2
F_TSTLK = 0xf
F_ULOCK = 0x0
F_UNLCK = 0x3
F_WRLCK = 0x2
HUPCL = 0x400
IBSHIFT = 0x10
ICANON = 0x2
ICMP6_FILTER = 0x26
ICMP6_SEC_SEND_DEL = 0x46
ICMP6_SEC_SEND_GET = 0x47
ICMP6_SEC_SEND_SET = 0x44
ICMP6_SEC_SEND_SET_CGA_ADDR = 0x45
ICRNL = 0x100
IEXTEN = 0x200000
IFA_FIRSTALIAS = 0x2000
IFA_ROUTE = 0x1
IFF_64BIT = 0x4000000
IFF_ALLCAST = 0x20000
IFF_ALLMULTI = 0x200
IFF_BPF = 0x8000000
IFF_BRIDGE = 0x40000
IFF_BROADCAST = 0x2
IFF_CANTCHANGE = 0x80c52
IFF_CHECKSUM_OFFLOAD = 0x10000000
IFF_D1 = 0x8000
IFF_D2 = 0x4000
IFF_D3 = 0x2000
IFF_D4 = 0x1000
IFF_DEBUG = 0x4
IFF_DEVHEALTH = 0x4000
IFF_DO_HW_LOOPBACK = 0x10000
IFF_GROUP_ROUTING = 0x2000000
IFF_IFBUFMGT = 0x800000
IFF_LINK0 = 0x100000
IFF_LINK1 = 0x200000
IFF_LINK2 = 0x400000
IFF_LOOPBACK = 0x8
IFF_MULTICAST = 0x80000
IFF_NOARP = 0x80
IFF_NOECHO = 0x800
IFF_NOTRAILERS = 0x20
IFF_OACTIVE = 0x400
IFF_POINTOPOINT = 0x10
IFF_PROMISC = 0x100
IFF_PSEG = 0x40000000
IFF_RUNNING = 0x40
IFF_SIMPLEX = 0x800
IFF_SNAP = 0x8000
IFF_TCP_DISABLE_CKSUM = 0x20000000
IFF_TCP_NOCKSUM = 0x1000000
IFF_UP = 0x1
IFF_VIPA = 0x80000000
IFNAMSIZ = 0x10
IFO_FLUSH = 0x1
IFT_1822 = 0x2
IFT_AAL5 = 0x31
IFT_ARCNET = 0x23
IFT_ARCNETPLUS = 0x24
IFT_ATM = 0x25
IFT_CEPT = 0x13
IFT_CLUSTER = 0x3e
IFT_DS3 = 0x1e
IFT_EON = 0x19
IFT_ETHER = 0x6
IFT_FCS = 0x3a
IFT_FDDI = 0xf
IFT_FRELAY = 0x20
IFT_FRELAYDCE = 0x2c
IFT_GIFTUNNEL = 0x3c
IFT_HDH1822 = 0x3
IFT_HF = 0x3d
IFT_HIPPI = 0x2f
IFT_HSSI = 0x2e
IFT_HY = 0xe
IFT_IB = 0xc7
IFT_ISDNBASIC = 0x14
IFT_ISDNPRIMARY = 0x15
IFT_ISO88022LLC = 0x29
IFT_ISO88023 = 0x7
IFT_ISO88024 = 0x8
IFT_ISO88025 = 0x9
IFT_ISO88026 = 0xa
IFT_LAPB = 0x10
IFT_LOCALTALK = 0x2a
IFT_LOOP = 0x18
IFT_MIOX25 = 0x26
IFT_MODEM = 0x30
IFT_NSIP = 0x1b
IFT_OTHER = 0x1
IFT_P10 = 0xc
IFT_P80 = 0xd
IFT_PARA = 0x22
IFT_PPP = 0x17
IFT_PROPMUX = 0x36
IFT_PROPVIRTUAL = 0x35
IFT_PTPSERIAL = 0x16
IFT_RS232 = 0x21
IFT_SDLC = 0x11
IFT_SIP = 0x1f
IFT_SLIP = 0x1c
IFT_SMDSDXI = 0x2b
IFT_SMDSICIP = 0x34
IFT_SN = 0x38
IFT_SONET = 0x27
IFT_SONETPATH = 0x32
IFT_SONETVT = 0x33
IFT_SP = 0x39
IFT_STARLAN = 0xb
IFT_T1 = 0x12
IFT_TUNNEL = 0x3b
IFT_ULTRA = 0x1d
IFT_V35 = 0x2d
IFT_VIPA = 0x37
IFT_X25 = 0x5
IFT_X25DDN = 0x4
IFT_X25PLE = 0x28
IFT_XETHER = 0x1a
IGNBRK = 0x1
IGNCR = 0x80
IGNPAR = 0x4
IMAXBEL = 0x10000
INLCR = 0x40
INPCK = 0x10
IN_CLASSA_HOST = 0xffffff
IN_CLASSA_MAX = 0x80
IN_CLASSA_NET = 0xff000000
IN_CLASSA_NSHIFT = 0x18
IN_CLASSB_HOST = 0xffff
IN_CLASSB_MAX = 0x10000
IN_CLASSB_NET = 0xffff0000
IN_CLASSB_NSHIFT = 0x10
IN_CLASSC_HOST = 0xff
IN_CLASSC_NET = 0xffffff00
IN_CLASSC_NSHIFT = 0x8
IN_CLASSD_HOST = 0xfffffff
IN_CLASSD_NET = 0xf0000000
IN_CLASSD_NSHIFT = 0x1c
IN_LOOPBACKNET = 0x7f
IN_USE = 0x1
IPPROTO_AH = 0x33
IPPROTO_BIP = 0x53
IPPROTO_DSTOPTS = 0x3c
IPPROTO_EGP = 0x8
IPPROTO_EON = 0x50
IPPROTO_ESP = 0x32
IPPROTO_FRAGMENT = 0x2c
IPPROTO_GGP = 0x3
IPPROTO_GIF = 0x8c
IPPROTO_GRE = 0x2f
IPPROTO_HOPOPTS = 0x0
IPPROTO_ICMP = 0x1
IPPROTO_ICMPV6 = 0x3a
IPPROTO_IDP = 0x16
IPPROTO_IGMP = 0x2
IPPROTO_IP = 0x0
IPPROTO_IPIP = 0x4
IPPROTO_IPV6 = 0x29
IPPROTO_LOCAL = 0x3f
IPPROTO_MAX = 0x100
IPPROTO_MH = 0x87
IPPROTO_NONE = 0x3b
IPPROTO_PUP = 0xc
IPPROTO_QOS = 0x2d
IPPROTO_RAW = 0xff
IPPROTO_ROUTING = 0x2b
IPPROTO_RSVP = 0x2e
IPPROTO_SCTP = 0x84
IPPROTO_TCP = 0x6
IPPROTO_TP = 0x1d
IPPROTO_UDP = 0x11
IPV6_ADDRFORM = 0x16
IPV6_ADDR_PREFERENCES = 0x4a
IPV6_ADD_MEMBERSHIP = 0xc
IPV6_AIXRAWSOCKET = 0x39
IPV6_CHECKSUM = 0x27
IPV6_DONTFRAG = 0x2d
IPV6_DROP_MEMBERSHIP = 0xd
IPV6_DSTOPTS = 0x36
IPV6_FLOWINFO_FLOWLABEL = 0xffffff
IPV6_FLOWINFO_PRIFLOW = 0xfffffff
IPV6_FLOWINFO_PRIORITY = 0xf000000
IPV6_FLOWINFO_SRFLAG = 0x10000000
IPV6_FLOWINFO_VERSION = 0xf0000000
IPV6_HOPLIMIT = 0x28
IPV6_HOPOPTS = 0x34
IPV6_JOIN_GROUP = 0xc
IPV6_LEAVE_GROUP = 0xd
IPV6_MIPDSTOPTS = 0x36
IPV6_MULTICAST_HOPS = 0xa
IPV6_MULTICAST_IF = 0x9
IPV6_MULTICAST_LOOP = 0xb
IPV6_NEXTHOP = 0x30
IPV6_NOPROBE = 0x1c
IPV6_PATHMTU = 0x2e
IPV6_PKTINFO = 0x21
IPV6_PKTOPTIONS = 0x24
IPV6_PRIORITY_10 = 0xa000000
IPV6_PRIORITY_11 = 0xb000000
IPV6_PRIORITY_12 = 0xc000000
IPV6_PRIORITY_13 = 0xd000000
IPV6_PRIORITY_14 = 0xe000000
IPV6_PRIORITY_15 = 0xf000000
IPV6_PRIORITY_8 = 0x8000000
IPV6_PRIORITY_9 = 0x9000000
IPV6_PRIORITY_BULK = 0x4000000
IPV6_PRIORITY_CONTROL = 0x7000000
IPV6_PRIORITY_FILLER = 0x1000000
IPV6_PRIORITY_INTERACTIVE = 0x6000000
IPV6_PRIORITY_RESERVED1 = 0x3000000
IPV6_PRIORITY_RESERVED2 = 0x5000000
IPV6_PRIORITY_UNATTENDED = 0x2000000
IPV6_PRIORITY_UNCHARACTERIZED = 0x0
IPV6_RECVDSTOPTS = 0x38
IPV6_RECVHOPLIMIT = 0x29
IPV6_RECVHOPOPTS = 0x35
IPV6_RECVHOPS = 0x22
IPV6_RECVIF = 0x1e
IPV6_RECVPATHMTU = 0x2f
IPV6_RECVPKTINFO = 0x23
IPV6_RECVRTHDR = 0x33
IPV6_RECVSRCRT = 0x1d
IPV6_RECVTCLASS = 0x2a
IPV6_RTHDR = 0x32
IPV6_RTHDRDSTOPTS = 0x37
IPV6_RTHDR_TYPE_0 = 0x0
IPV6_RTHDR_TYPE_2 = 0x2
IPV6_SENDIF = 0x1f
IPV6_SRFLAG_LOOSE = 0x0
IPV6_SRFLAG_STRICT = 0x10000000
IPV6_TCLASS = 0x2b
IPV6_TOKEN_LENGTH = 0x40
IPV6_UNICAST_HOPS = 0x4
IPV6_USE_MIN_MTU = 0x2c
IPV6_V6ONLY = 0x25
IPV6_VERSION = 0x60000000
IP_ADDRFORM = 0x16
IP_ADD_MEMBERSHIP = 0xc
IP_ADD_SOURCE_MEMBERSHIP = 0x3c
IP_BLOCK_SOURCE = 0x3a
IP_BROADCAST_IF = 0x10
IP_CACHE_LINE_SIZE = 0x80
IP_DEFAULT_MULTICAST_LOOP = 0x1
IP_DEFAULT_MULTICAST_TTL = 0x1
IP_DF = 0x4000
IP_DHCPMODE = 0x11
IP_DONTFRAG = 0x19
IP_DROP_MEMBERSHIP = 0xd
IP_DROP_SOURCE_MEMBERSHIP = 0x3d
IP_FINDPMTU = 0x1a
IP_HDRINCL = 0x2
IP_INC_MEMBERSHIPS = 0x14
IP_INIT_MEMBERSHIP = 0x14
IP_MAXPACKET = 0xffff
IP_MF = 0x2000
IP_MSS = 0x240
IP_MULTICAST_HOPS = 0xa
IP_MULTICAST_IF = 0x9
IP_MULTICAST_LOOP = 0xb
IP_MULTICAST_TTL = 0xa
IP_OPT = 0x1b
IP_OPTIONS = 0x1
IP_PMTUAGE = 0x1b
IP_RECVDSTADDR = 0x7
IP_RECVIF = 0x14
IP_RECVIFINFO = 0xf
IP_RECVINTERFACE = 0x20
IP_RECVMACHDR = 0xe
IP_RECVOPTS = 0x5
IP_RECVRETOPTS = 0x6
IP_RECVTTL = 0x22
IP_RETOPTS = 0x8
IP_SOURCE_FILTER = 0x48
IP_TOS = 0x3
IP_TTL = 0x4
IP_UNBLOCK_SOURCE = 0x3b
IP_UNICAST_HOPS = 0x4
ISIG = 0x1
ISTRIP = 0x20
IUCLC = 0x800
IXANY = 0x1000
IXOFF = 0x400
IXON = 0x200
I_FLUSH = 0x20005305
LNOFLSH = 0x8000
LOCK_EX = 0x2
LOCK_NB = 0x4
LOCK_SH = 0x1
LOCK_UN = 0x8
MADV_DONTNEED = 0x4
MADV_NORMAL = 0x0
MADV_RANDOM = 0x1
MADV_SEQUENTIAL = 0x2
MADV_SPACEAVAIL = 0x5
MADV_WILLNEED = 0x3
MAP_ANON = 0x10
MAP_ANONYMOUS = 0x10
MAP_FILE = 0x0
MAP_FIXED = 0x100
MAP_PRIVATE = 0x2
MAP_SHARED = 0x1
MAP_TYPE = 0xf0
MAP_VARIABLE = 0x0
MCAST_BLOCK_SOURCE = 0x40
MCAST_EXCLUDE = 0x2
MCAST_INCLUDE = 0x1
MCAST_JOIN_GROUP = 0x3e
MCAST_JOIN_SOURCE_GROUP = 0x42
MCAST_LEAVE_GROUP = 0x3f
MCAST_LEAVE_SOURCE_GROUP = 0x43
MCAST_SOURCE_FILTER = 0x49
MCAST_UNBLOCK_SOURCE = 0x41
MCL_CURRENT = 0x100
MCL_FUTURE = 0x200
MSG_ANY = 0x4
MSG_ARGEXT = 0x400
MSG_BAND = 0x2
MSG_COMPAT = 0x8000
MSG_CTRUNC = 0x20
MSG_DONTROUTE = 0x4
MSG_EOR = 0x8
MSG_HIPRI = 0x1
MSG_MAXIOVLEN = 0x10
MSG_MPEG2 = 0x80
MSG_NONBLOCK = 0x4000
MSG_NOSIGNAL = 0x100
MSG_OOB = 0x1
MSG_PEEK = 0x2
MSG_TRUNC = 0x10
MSG_WAITALL = 0x40
MSG_WAITFORONE = 0x200
MS_ASYNC = 0x10
MS_EINTR = 0x80
MS_INVALIDATE = 0x40
MS_PER_SEC = 0x3e8
MS_SYNC = 0x20
NFDBITS = 0x40
NL0 = 0x0
NL1 = 0x4000
NL2 = 0x8000
NL3 = 0xc000
NLDLY = 0x4000
NOFLSH = 0x80
NOFLUSH = 0x80000000
OCRNL = 0x8
OFDEL = 0x80
OFILL = 0x40
OLCUC = 0x2
ONLCR = 0x4
ONLRET = 0x20
ONOCR = 0x10
ONOEOT = 0x80000
OPOST = 0x1
OXTABS = 0x40000
O_ACCMODE = 0x23
O_APPEND = 0x8
O_CIO = 0x80
O_CIOR = 0x800000000
O_CLOEXEC = 0x800000
O_CREAT = 0x100
O_DEFER = 0x2000
O_DELAY = 0x4000
O_DIRECT = 0x8000000
O_DIRECTORY = 0x80000
O_DSYNC = 0x400000
O_EFSOFF = 0x400000000
O_EFSON = 0x200000000
O_EXCL = 0x400
O_EXEC = 0x20
O_LARGEFILE = 0x4000000
O_NDELAY = 0x8000
O_NOCACHE = 0x100000
O_NOCTTY = 0x800
O_NOFOLLOW = 0x1000000
O_NONBLOCK = 0x4
O_NONE = 0x3
O_NSHARE = 0x10000
O_RAW = 0x100000000
O_RDONLY = 0x0
O_RDWR = 0x2
O_RSHARE = 0x1000
O_RSYNC = 0x200000
O_SEARCH = 0x20
O_SNAPSHOT = 0x40
O_SYNC = 0x10
O_TRUNC = 0x200
O_TTY_INIT = 0x0
O_WRONLY = 0x1
PARENB = 0x100
PAREXT = 0x100000
PARMRK = 0x8
PARODD = 0x200
PENDIN = 0x20000000
PRIO_PGRP = 0x1
PRIO_PROCESS = 0x0
PRIO_USER = 0x2
PROT_EXEC = 0x4
PROT_NONE = 0x0
PROT_READ = 0x1
PROT_WRITE = 0x2
PR_64BIT = 0x20
PR_ADDR = 0x2
PR_ARGEXT = 0x400
PR_ATOMIC = 0x1
PR_CONNREQUIRED = 0x4
PR_FASTHZ = 0x5
PR_INP = 0x40
PR_INTRLEVEL = 0x8000
PR_MLS = 0x100
PR_MLS_1_LABEL = 0x200
PR_NOEOR = 0x4000
PR_RIGHTS = 0x10
PR_SLOWHZ = 0x2
PR_WANTRCVD = 0x8
RLIMIT_AS = 0x6
RLIMIT_CORE = 0x4
RLIMIT_CPU = 0x0
RLIMIT_DATA = 0x2
RLIMIT_FSIZE = 0x1
RLIMIT_NOFILE = 0x7
RLIMIT_NPROC = 0x9
RLIMIT_RSS = 0x5
RLIMIT_STACK = 0x3
RLIM_INFINITY = 0x7fffffffffffffff
RTAX_AUTHOR = 0x6
RTAX_BRD = 0x7
RTAX_DST = 0x0
RTAX_GATEWAY = 0x1
RTAX_GENMASK = 0x3
RTAX_IFA = 0x5
RTAX_IFP = 0x4
RTAX_MAX = 0x8
RTAX_NETMASK = 0x2
RTA_AUTHOR = 0x40
RTA_BRD = 0x80
RTA_DOWNSTREAM = 0x100
RTA_DST = 0x1
RTA_GATEWAY = 0x2
RTA_GENMASK = 0x8
RTA_IFA = 0x20
RTA_IFP = 0x10
RTA_NETMASK = 0x4
RTC_IA64 = 0x3
RTC_POWER = 0x1
RTC_POWER_PC = 0x2
RTF_ACTIVE_DGD = 0x1000000
RTF_BCE = 0x80000
RTF_BLACKHOLE = 0x1000
RTF_BROADCAST = 0x400000
RTF_BUL = 0x2000
RTF_CLONE = 0x10000
RTF_CLONED = 0x20000
RTF_CLONING = 0x100
RTF_DONE = 0x40
RTF_DYNAMIC = 0x10
RTF_FREE_IN_PROG = 0x4000000
RTF_GATEWAY = 0x2
RTF_HOST = 0x4
RTF_LLINFO = 0x400
RTF_LOCAL = 0x200000
RTF_MASK = 0x80
RTF_MODIFIED = 0x20
RTF_MULTICAST = 0x800000
RTF_PERMANENT6 = 0x8000000
RTF_PINNED = 0x100000
RTF_PROTO1 = 0x8000
RTF_PROTO2 = 0x4000
RTF_PROTO3 = 0x40000
RTF_REJECT = 0x8
RTF_SMALLMTU = 0x40000
RTF_STATIC = 0x800
RTF_STOPSRCH = 0x2000000
RTF_UNREACHABLE = 0x10000000
RTF_UP = 0x1
RTF_XRESOLVE = 0x200
RTM_ADD = 0x1
RTM_CHANGE = 0x3
RTM_DELADDR = 0xd
RTM_DELETE = 0x2
RTM_EXPIRE = 0xf
RTM_GET = 0x4
RTM_GETNEXT = 0x11
RTM_IFINFO = 0xe
RTM_LOCK = 0x8
RTM_LOSING = 0x5
RTM_MISS = 0x7
RTM_NEWADDR = 0xc
RTM_OLDADD = 0x9
RTM_OLDDEL = 0xa
RTM_REDIRECT = 0x6
RTM_RESOLVE = 0xb
RTM_RTLOST = 0x10
RTM_RTTUNIT = 0xf4240
RTM_SAMEADDR = 0x12
RTM_SET = 0x13
RTM_VERSION = 0x2
RTM_VERSION_GR = 0x4
RTM_VERSION_GR_COMPAT = 0x3
RTM_VERSION_POLICY = 0x5
RTM_VERSION_POLICY_EXT = 0x6
RTM_VERSION_POLICY_PRFN = 0x7
RTV_EXPIRE = 0x4
RTV_HOPCOUNT = 0x2
RTV_MTU = 0x1
RTV_RPIPE = 0x8
RTV_RTT = 0x40
RTV_RTTVAR = 0x80
RTV_SPIPE = 0x10
RTV_SSTHRESH = 0x20
RUSAGE_CHILDREN = -0x1
RUSAGE_SELF = 0x0
RUSAGE_THREAD = 0x1
SCM_RIGHTS = 0x1
SHUT_RD = 0x0
SHUT_RDWR = 0x2
SHUT_WR = 0x1
SIGMAX64 = 0xff
SIGQUEUE_MAX = 0x20
SIOCADDIFVIPA = 0x20006942
SIOCADDMTU = -0x7ffb9690
SIOCADDMULTI = -0x7fdf96cf
SIOCADDNETID = -0x7fd796a9
SIOCADDRT = -0x7fc78df6
SIOCAIFADDR = -0x7fbf96e6
SIOCATMARK = 0x40047307
SIOCDARP = -0x7fb396e0
SIOCDELIFVIPA = 0x20006943
SIOCDELMTU = -0x7ffb968f
SIOCDELMULTI = -0x7fdf96ce
SIOCDELPMTU = -0x7fd78ff6
SIOCDELRT = -0x7fc78df5
SIOCDIFADDR = -0x7fd796e7
SIOCDNETOPT = -0x3ffe9680
SIOCDX25XLATE = -0x7fd7969b
SIOCFIFADDR = -0x7fdf966d
SIOCGARP = -0x3fb396da
SIOCGETMTUS = 0x2000696f
SIOCGETSGCNT = -0x3feb8acc
SIOCGETVIFCNT = -0x3feb8acd
SIOCGHIWAT = 0x40047301
SIOCGIFADDR = -0x3fd796df
SIOCGIFADDRS = 0x2000698c
SIOCGIFBAUDRATE = -0x3fdf9669
SIOCGIFBRDADDR = -0x3fd796dd
SIOCGIFCONF = -0x3fef96bb
SIOCGIFCONFGLOB = -0x3fef9670
SIOCGIFDSTADDR = -0x3fd796de
SIOCGIFFLAGS = -0x3fd796ef
SIOCGIFGIDLIST = 0x20006968
SIOCGIFHWADDR = -0x3fab966b
SIOCGIFMETRIC = -0x3fd796e9
SIOCGIFMTU = -0x3fd796aa
SIOCGIFNETMASK = -0x3fd796db
SIOCGIFOPTIONS = -0x3fd796d6
SIOCGISNO = -0x3fd79695
SIOCGLOADF = -0x3ffb967e
SIOCGLOWAT = 0x40047303
SIOCGNETOPT = -0x3ffe96a5
SIOCGNETOPT1 = -0x3fdf967f
SIOCGNMTUS = 0x2000696e
SIOCGPGRP = 0x40047309
SIOCGSIZIFCONF = 0x4004696a
SIOCGSRCFILTER = -0x3fe796cb
SIOCGTUNEPHASE = -0x3ffb9676
SIOCGX25XLATE = -0x3fd7969c
SIOCIFATTACH = -0x7fdf9699
SIOCIFDETACH = -0x7fdf969a
SIOCIFGETPKEY = -0x7fdf969b
SIOCIF_ATM_DARP = -0x7fdf9683
SIOCIF_ATM_DUMPARP = -0x7fdf9685
SIOCIF_ATM_GARP = -0x7fdf9682
SIOCIF_ATM_IDLE = -0x7fdf9686
SIOCIF_ATM_SARP = -0x7fdf9681
SIOCIF_ATM_SNMPARP = -0x7fdf9687
SIOCIF_ATM_SVC = -0x7fdf9684
SIOCIF_ATM_UBR = -0x7fdf9688
SIOCIF_DEVHEALTH = -0x7ffb966c
SIOCIF_IB_ARP_INCOMP = -0x7fdf9677
SIOCIF_IB_ARP_TIMER = -0x7fdf9678
SIOCIF_IB_CLEAR_PINFO = -0x3fdf966f
SIOCIF_IB_DEL_ARP = -0x7fdf967f
SIOCIF_IB_DEL_PINFO = -0x3fdf9670
SIOCIF_IB_DUMP_ARP = -0x7fdf9680
SIOCIF_IB_GET_ARP = -0x7fdf967e
SIOCIF_IB_GET_INFO = -0x3f879675
SIOCIF_IB_GET_STATS = -0x3f879672
SIOCIF_IB_NOTIFY_ADDR_REM = -0x3f87966a
SIOCIF_IB_RESET_STATS = -0x3f879671
SIOCIF_IB_RESIZE_CQ = -0x7fdf9679
SIOCIF_IB_SET_ARP = -0x7fdf967d
SIOCIF_IB_SET_PKEY = -0x7fdf967c
SIOCIF_IB_SET_PORT = -0x7fdf967b
SIOCIF_IB_SET_QKEY = -0x7fdf9676
SIOCIF_IB_SET_QSIZE = -0x7fdf967a
SIOCLISTIFVIPA = 0x20006944
SIOCSARP = -0x7fb396e2
SIOCSHIWAT = 0xffffffff80047300
SIOCSIFADDR = -0x7fd796f4
SIOCSIFADDRORI = -0x7fdb9673
SIOCSIFBRDADDR = -0x7fd796ed
SIOCSIFDSTADDR = -0x7fd796f2
SIOCSIFFLAGS = -0x7fd796f0
SIOCSIFGIDLIST = 0x20006969
SIOCSIFMETRIC = -0x7fd796e8
SIOCSIFMTU = -0x7fd796a8
SIOCSIFNETDUMP = -0x7fd796e4
SIOCSIFNETMASK = -0x7fd796ea
SIOCSIFOPTIONS = -0x7fd796d7
SIOCSIFSUBCHAN = -0x7fd796e5
SIOCSISNO = -0x7fd79694
SIOCSLOADF = -0x3ffb967d
SIOCSLOWAT = 0xffffffff80047302
SIOCSNETOPT = -0x7ffe96a6
SIOCSPGRP = 0xffffffff80047308
SIOCSX25XLATE = -0x7fd7969d
SOCK_CONN_DGRAM = 0x6
SOCK_DGRAM = 0x2
SOCK_RAW = 0x3
SOCK_RDM = 0x4
SOCK_SEQPACKET = 0x5
SOCK_STREAM = 0x1
SOL_SOCKET = 0xffff
SOMAXCONN = 0x400
SO_ACCEPTCONN = 0x2
SO_AUDIT = 0x8000
SO_BROADCAST = 0x20
SO_CKSUMRECV = 0x800
SO_DEBUG = 0x1
SO_DONTROUTE = 0x10
SO_ERROR = 0x1007
SO_KEEPALIVE = 0x8
SO_KERNACCEPT = 0x2000
SO_LINGER = 0x80
SO_NOMULTIPATH = 0x4000
SO_NOREUSEADDR = 0x1000
SO_OOBINLINE = 0x100
SO_PEERID = 0x1009
SO_RCVBUF = 0x1002
SO_RCVLOWAT = 0x1004
SO_RCVTIMEO = 0x1006
SO_REUSEADDR = 0x4
SO_REUSEPORT = 0x200
SO_SNDBUF = 0x1001
SO_SNDLOWAT = 0x1003
SO_SNDTIMEO = 0x1005
SO_TIMESTAMPNS = 0x100a
SO_TYPE = 0x1008
SO_USELOOPBACK = 0x40
SO_USE_IFBUFS = 0x400
S_BANDURG = 0x400
S_EMODFMT = 0x3c000000
S_ENFMT = 0x400
S_ERROR = 0x100
S_HANGUP = 0x200
S_HIPRI = 0x2
S_ICRYPTO = 0x80000
S_IEXEC = 0x40
S_IFBLK = 0x6000
S_IFCHR = 0x2000
S_IFDIR = 0x4000
S_IFIFO = 0x1000
S_IFJOURNAL = 0x10000
S_IFLNK = 0xa000
S_IFMPX = 0x2200
S_IFMT = 0xf000
S_IFPDIR = 0x4000000
S_IFPSDIR = 0x8000000
S_IFPSSDIR = 0xc000000
S_IFREG = 0x8000
S_IFSOCK = 0xc000
S_IFSYSEA = 0x30000000
S_INPUT = 0x1
S_IREAD = 0x100
S_IRGRP = 0x20
S_IROTH = 0x4
S_IRUSR = 0x100
S_IRWXG = 0x38
S_IRWXO = 0x7
S_IRWXU = 0x1c0
S_ISGID = 0x400
S_ISUID = 0x800
S_ISVTX = 0x200
S_ITCB = 0x1000000
S_ITP = 0x800000
S_IWGRP = 0x10
S_IWOTH = 0x2
S_IWRITE = 0x80
S_IWUSR = 0x80
S_IXACL = 0x2000000
S_IXATTR = 0x40000
S_IXGRP = 0x8
S_IXINTERFACE = 0x100000
S_IXMOD = 0x40000000
S_IXOTH = 0x1
S_IXUSR = 0x40
S_MSG = 0x8
S_OUTPUT = 0x4
S_RDBAND = 0x20
S_RDNORM = 0x10
S_RESERVED1 = 0x20000
S_RESERVED2 = 0x200000
S_RESERVED3 = 0x400000
S_RESERVED4 = 0x80000000
S_RESFMT1 = 0x10000000
S_RESFMT10 = 0x34000000
S_RESFMT11 = 0x38000000
S_RESFMT12 = 0x3c000000
S_RESFMT2 = 0x14000000
S_RESFMT3 = 0x18000000
S_RESFMT4 = 0x1c000000
S_RESFMT5 = 0x20000000
S_RESFMT6 = 0x24000000
S_RESFMT7 = 0x28000000
S_RESFMT8 = 0x2c000000
S_WRBAND = 0x80
S_WRNORM = 0x40
TAB0 = 0x0
TAB1 = 0x400
TAB2 = 0x800
TAB3 = 0xc00
TABDLY = 0xc00
TCFLSH = 0x540c
TCGETA = 0x5405
TCGETS = 0x5401
TCIFLUSH = 0x0
TCIOFF = 0x2
TCIOFLUSH = 0x2
TCION = 0x3
TCOFLUSH = 0x1
TCOOFF = 0x0
TCOON = 0x1
TCP_24DAYS_WORTH_OF_SLOWTICKS = 0x3f4800
TCP_ACLADD = 0x23
TCP_ACLBIND = 0x26
TCP_ACLCLEAR = 0x22
TCP_ACLDEL = 0x24
TCP_ACLDENY = 0x8
TCP_ACLFLUSH = 0x21
TCP_ACLGID = 0x1
TCP_ACLLS = 0x25
TCP_ACLSUBNET = 0x4
TCP_ACLUID = 0x2
TCP_CWND_DF = 0x16
TCP_CWND_IF = 0x15
TCP_DELAY_ACK_FIN = 0x2
TCP_DELAY_ACK_SYN = 0x1
TCP_FASTNAME = 0x101080a
TCP_KEEPCNT = 0x13
TCP_KEEPIDLE = 0x11
TCP_KEEPINTVL = 0x12
TCP_LSPRIV = 0x29
TCP_LUID = 0x20
TCP_MAXBURST = 0x8
TCP_MAXDF = 0x64
TCP_MAXIF = 0x64
TCP_MAXSEG = 0x2
TCP_MAXWIN = 0xffff
TCP_MAXWINDOWSCALE = 0xe
TCP_MAX_SACK = 0x4
TCP_MSS = 0x5b4
TCP_NODELAY = 0x1
TCP_NODELAYACK = 0x14
TCP_NOREDUCE_CWND_EXIT_FRXMT = 0x19
TCP_NOREDUCE_CWND_IN_FRXMT = 0x18
TCP_NOTENTER_SSTART = 0x17
TCP_OPT = 0x19
TCP_RFC1323 = 0x4
TCP_SETPRIV = 0x27
TCP_STDURG = 0x10
TCP_TIMESTAMP_OPTLEN = 0xc
TCP_UNSETPRIV = 0x28
TCSAFLUSH = 0x2
TCSBRK = 0x5409
TCSETA = 0x5406
TCSETAF = 0x5408
TCSETAW = 0x5407
TCSETS = 0x5402
TCSETSF = 0x5404
TCSETSW = 0x5403
TCXONC = 0x540b
TIMER_ABSTIME = 0x3e7
TIMER_MAX = 0x20
TIOC = 0x5400
TIOCCBRK = 0x2000747a
TIOCCDTR = 0x20007478
TIOCCONS = 0xffffffff80047462
TIOCEXCL = 0x2000740d
TIOCFLUSH = 0xffffffff80047410
TIOCGETC = 0x40067412
TIOCGETD = 0x40047400
TIOCGETP = 0x40067408
TIOCGLTC = 0x40067474
TIOCGPGRP = 0x40047477
TIOCGSID = 0x40047448
TIOCGSIZE = 0x40087468
TIOCGWINSZ = 0x40087468
TIOCHPCL = 0x20007402
TIOCLBIC = 0xffffffff8004747e
TIOCLBIS = 0xffffffff8004747f
TIOCLGET = 0x4004747c
TIOCLSET = 0xffffffff8004747d
TIOCMBIC = 0xffffffff8004746b
TIOCMBIS = 0xffffffff8004746c
TIOCMGET = 0x4004746a
TIOCMIWAIT = 0xffffffff80047464
TIOCMODG = 0x40047403
TIOCMODS = 0xffffffff80047404
TIOCMSET = 0xffffffff8004746d
TIOCM_CAR = 0x40
TIOCM_CD = 0x40
TIOCM_CTS = 0x20
TIOCM_DSR = 0x100
TIOCM_DTR = 0x2
TIOCM_LE = 0x1
TIOCM_RI = 0x80
TIOCM_RNG = 0x80
TIOCM_RTS = 0x4
TIOCM_SR = 0x10
TIOCM_ST = 0x8
TIOCNOTTY = 0x20007471
TIOCNXCL = 0x2000740e
TIOCOUTQ = 0x40047473
TIOCPKT = 0xffffffff80047470
TIOCPKT_DATA = 0x0
TIOCPKT_DOSTOP = 0x20
TIOCPKT_FLUSHREAD = 0x1
TIOCPKT_FLUSHWRITE = 0x2
TIOCPKT_NOSTOP = 0x10
TIOCPKT_START = 0x8
TIOCPKT_STOP = 0x4
TIOCREMOTE = 0xffffffff80047469
TIOCSBRK = 0x2000747b
TIOCSDTR = 0x20007479
TIOCSETC = 0xffffffff80067411
TIOCSETD = 0xffffffff80047401
TIOCSETN = 0xffffffff8006740a
TIOCSETP = 0xffffffff80067409
TIOCSLTC = 0xffffffff80067475
TIOCSPGRP = 0xffffffff80047476
TIOCSSIZE = 0xffffffff80087467
TIOCSTART = 0x2000746e
TIOCSTI = 0xffffffff80017472
TIOCSTOP = 0x2000746f
TIOCSWINSZ = 0xffffffff80087467
TIOCUCNTL = 0xffffffff80047466
TOSTOP = 0x10000
UTIME_NOW = -0x2
UTIME_OMIT = -0x3
VDISCRD = 0xc
VDSUSP = 0xa
VEOF = 0x4
VEOL = 0x5
VEOL2 = 0x6
VERASE = 0x2
VINTR = 0x0
VKILL = 0x3
VLNEXT = 0xe
VMIN = 0x4
VQUIT = 0x1
VREPRINT = 0xb
VSTART = 0x7
VSTOP = 0x8
VSTRT = 0x7
VSUSP = 0x9
VT0 = 0x0
VT1 = 0x8000
VTDELAY = 0x2000
VTDLY = 0x8000
VTIME = 0x5
VWERSE = 0xd
WPARSTART = 0x1
WPARSTOP = 0x2
WPARTTYNAME = "Global"
XCASE = 0x4
XTABS = 0xc00
_FDATAFLUSH = 0x2000000000
)
// Errors
const (
E2BIG = syscall.Errno(0x7)
EACCES = syscall.Errno(0xd)
EADDRINUSE = syscall.Errno(0x43)
EADDRNOTAVAIL = syscall.Errno(0x44)
EAFNOSUPPORT = syscall.Errno(0x42)
EAGAIN = syscall.Errno(0xb)
EALREADY = syscall.Errno(0x38)
EBADF = syscall.Errno(0x9)
EBADMSG = syscall.Errno(0x78)
EBUSY = syscall.Errno(0x10)
ECANCELED = syscall.Errno(0x75)
ECHILD = syscall.Errno(0xa)
ECHRNG = syscall.Errno(0x25)
ECLONEME = syscall.Errno(0x52)
ECONNABORTED = syscall.Errno(0x48)
ECONNREFUSED = syscall.Errno(0x4f)
ECONNRESET = syscall.Errno(0x49)
ECORRUPT = syscall.Errno(0x59)
EDEADLK = syscall.Errno(0x2d)
EDESTADDREQ = syscall.Errno(0x3a)
EDESTADDRREQ = syscall.Errno(0x3a)
EDIST = syscall.Errno(0x35)
EDOM = syscall.Errno(0x21)
EDQUOT = syscall.Errno(0x58)
EEXIST = syscall.Errno(0x11)
EFAULT = syscall.Errno(0xe)
EFBIG = syscall.Errno(0x1b)
EFORMAT = syscall.Errno(0x30)
EHOSTDOWN = syscall.Errno(0x50)
EHOSTUNREACH = syscall.Errno(0x51)
EIDRM = syscall.Errno(0x24)
EILSEQ = syscall.Errno(0x74)
EINPROGRESS = syscall.Errno(0x37)
EINTR = syscall.Errno(0x4)
EINVAL = syscall.Errno(0x16)
EIO = syscall.Errno(0x5)
EISCONN = syscall.Errno(0x4b)
EISDIR = syscall.Errno(0x15)
EL2HLT = syscall.Errno(0x2c)
EL2NSYNC = syscall.Errno(0x26)
EL3HLT = syscall.Errno(0x27)
EL3RST = syscall.Errno(0x28)
ELNRNG = syscall.Errno(0x29)
ELOOP = syscall.Errno(0x55)
EMEDIA = syscall.Errno(0x6e)
EMFILE = syscall.Errno(0x18)
EMLINK = syscall.Errno(0x1f)
EMSGSIZE = syscall.Errno(0x3b)
EMULTIHOP = syscall.Errno(0x7d)
ENAMETOOLONG = syscall.Errno(0x56)
ENETDOWN = syscall.Errno(0x45)
ENETRESET = syscall.Errno(0x47)
ENETUNREACH = syscall.Errno(0x46)
ENFILE = syscall.Errno(0x17)
ENOATTR = syscall.Errno(0x70)
ENOBUFS = syscall.Errno(0x4a)
ENOCONNECT = syscall.Errno(0x32)
ENOCSI = syscall.Errno(0x2b)
ENODATA = syscall.Errno(0x7a)
ENODEV = syscall.Errno(0x13)
ENOENT = syscall.Errno(0x2)
ENOEXEC = syscall.Errno(0x8)
ENOLCK = syscall.Errno(0x31)
ENOLINK = syscall.Errno(0x7e)
ENOMEM = syscall.Errno(0xc)
ENOMSG = syscall.Errno(0x23)
ENOPROTOOPT = syscall.Errno(0x3d)
ENOSPC = syscall.Errno(0x1c)
ENOSR = syscall.Errno(0x76)
ENOSTR = syscall.Errno(0x7b)
ENOSYS = syscall.Errno(0x6d)
ENOTBLK = syscall.Errno(0xf)
ENOTCONN = syscall.Errno(0x4c)
ENOTDIR = syscall.Errno(0x14)
ENOTEMPTY = syscall.Errno(0x11)
ENOTREADY = syscall.Errno(0x2e)
ENOTRECOVERABLE = syscall.Errno(0x5e)
ENOTRUST = syscall.Errno(0x72)
ENOTSOCK = syscall.Errno(0x39)
ENOTSUP = syscall.Errno(0x7c)
ENOTTY = syscall.Errno(0x19)
ENXIO = syscall.Errno(0x6)
EOPNOTSUPP = syscall.Errno(0x40)
EOVERFLOW = syscall.Errno(0x7f)
EOWNERDEAD = syscall.Errno(0x5f)
EPERM = syscall.Errno(0x1)
EPFNOSUPPORT = syscall.Errno(0x41)
EPIPE = syscall.Errno(0x20)
EPROCLIM = syscall.Errno(0x53)
EPROTO = syscall.Errno(0x79)
EPROTONOSUPPORT = syscall.Errno(0x3e)
EPROTOTYPE = syscall.Errno(0x3c)
ERANGE = syscall.Errno(0x22)
EREMOTE = syscall.Errno(0x5d)
ERESTART = syscall.Errno(0x52)
EROFS = syscall.Errno(0x1e)
ESAD = syscall.Errno(0x71)
ESHUTDOWN = syscall.Errno(0x4d)
ESOCKTNOSUPPORT = syscall.Errno(0x3f)
ESOFT = syscall.Errno(0x6f)
ESPIPE = syscall.Errno(0x1d)
ESRCH = syscall.Errno(0x3)
ESTALE = syscall.Errno(0x34)
ESYSERROR = syscall.Errno(0x5a)
ETIME = syscall.Errno(0x77)
ETIMEDOUT = syscall.Errno(0x4e)
ETOOMANYREFS = syscall.Errno(0x73)
ETXTBSY = syscall.Errno(0x1a)
EUNATCH = syscall.Errno(0x2a)
EUSERS = syscall.Errno(0x54)
EWOULDBLOCK = syscall.Errno(0xb)
EWRPROTECT = syscall.Errno(0x2f)
EXDEV = syscall.Errno(0x12)
)
// Signals
const (
SIGABRT = syscall.Signal(0x6)
SIGAIO = syscall.Signal(0x17)
SIGALRM = syscall.Signal(0xe)
SIGALRM1 = syscall.Signal(0x26)
SIGBUS = syscall.Signal(0xa)
SIGCAPI = syscall.Signal(0x31)
SIGCHLD = syscall.Signal(0x14)
SIGCLD = syscall.Signal(0x14)
SIGCONT = syscall.Signal(0x13)
SIGCPUFAIL = syscall.Signal(0x3b)
SIGDANGER = syscall.Signal(0x21)
SIGEMT = syscall.Signal(0x7)
SIGFPE = syscall.Signal(0x8)
SIGGRANT = syscall.Signal(0x3c)
SIGHUP = syscall.Signal(0x1)
SIGILL = syscall.Signal(0x4)
SIGINT = syscall.Signal(0x2)
SIGIO = syscall.Signal(0x17)
SIGIOINT = syscall.Signal(0x10)
SIGIOT = syscall.Signal(0x6)
SIGKAP = syscall.Signal(0x3c)
SIGKILL = syscall.Signal(0x9)
SIGLOST = syscall.Signal(0x6)
SIGMAX = syscall.Signal(0xff)
SIGMAX32 = syscall.Signal(0x3f)
SIGMIGRATE = syscall.Signal(0x23)
SIGMSG = syscall.Signal(0x1b)
SIGPIPE = syscall.Signal(0xd)
SIGPOLL = syscall.Signal(0x17)
SIGPRE = syscall.Signal(0x24)
SIGPROF = syscall.Signal(0x20)
SIGPTY = syscall.Signal(0x17)
SIGPWR = syscall.Signal(0x1d)
SIGQUIT = syscall.Signal(0x3)
SIGRECONFIG = syscall.Signal(0x3a)
SIGRETRACT = syscall.Signal(0x3d)
SIGSAK = syscall.Signal(0x3f)
SIGSEGV = syscall.Signal(0xb)
SIGSOUND = syscall.Signal(0x3e)
SIGSTOP = syscall.Signal(0x11)
SIGSYS = syscall.Signal(0xc)
SIGSYSERROR = syscall.Signal(0x30)
SIGTALRM = syscall.Signal(0x26)
SIGTERM = syscall.Signal(0xf)
SIGTRAP = syscall.Signal(0x5)
SIGTSTP = syscall.Signal(0x12)
SIGTTIN = syscall.Signal(0x15)
SIGTTOU = syscall.Signal(0x16)
SIGURG = syscall.Signal(0x10)
SIGUSR1 = syscall.Signal(0x1e)
SIGUSR2 = syscall.Signal(0x1f)
SIGVIRT = syscall.Signal(0x25)
SIGVTALRM = syscall.Signal(0x22)
SIGWAITING = syscall.Signal(0x27)
SIGWINCH = syscall.Signal(0x1c)
SIGXCPU = syscall.Signal(0x18)
SIGXFSZ = syscall.Signal(0x19)
)
// Error table
var errorList = [...]struct {
num syscall.Errno
name string
desc string
}{
{1, "EPERM", "not owner"},
{2, "ENOENT", "no such file or directory"},
{3, "ESRCH", "no such process"},
{4, "EINTR", "interrupted system call"},
{5, "EIO", "I/O error"},
{6, "ENXIO", "no such device or address"},
{7, "E2BIG", "arg list too long"},
{8, "ENOEXEC", "exec format error"},
{9, "EBADF", "bad file number"},
{10, "ECHILD", "no child processes"},
{11, "EWOULDBLOCK", "resource temporarily unavailable"},
{12, "ENOMEM", "not enough space"},
{13, "EACCES", "permission denied"},
{14, "EFAULT", "bad address"},
{15, "ENOTBLK", "block device required"},
{16, "EBUSY", "device busy"},
{17, "ENOTEMPTY", "file exists"},
{18, "EXDEV", "cross-device link"},
{19, "ENODEV", "no such device"},
{20, "ENOTDIR", "not a directory"},
{21, "EISDIR", "is a directory"},
{22, "EINVAL", "invalid argument"},
{23, "ENFILE", "file table overflow"},
{24, "EMFILE", "too many open files"},
{25, "ENOTTY", "not a typewriter"},
{26, "ETXTBSY", "text file busy"},
{27, "EFBIG", "file too large"},
{28, "ENOSPC", "no space left on device"},
{29, "ESPIPE", "illegal seek"},
{30, "EROFS", "read-only file system"},
{31, "EMLINK", "too many links"},
{32, "EPIPE", "broken pipe"},
{33, "EDOM", "argument out of domain"},
{34, "ERANGE", "result too large"},
{35, "ENOMSG", "no message of desired type"},
{36, "EIDRM", "identifier removed"},
{37, "ECHRNG", "channel number out of range"},
{38, "EL2NSYNC", "level 2 not synchronized"},
{39, "EL3HLT", "level 3 halted"},
{40, "EL3RST", "level 3 reset"},
{41, "ELNRNG", "link number out of range"},
{42, "EUNATCH", "protocol driver not attached"},
{43, "ENOCSI", "no CSI structure available"},
{44, "EL2HLT", "level 2 halted"},
{45, "EDEADLK", "deadlock condition if locked"},
{46, "ENOTREADY", "device not ready"},
{47, "EWRPROTECT", "write-protected media"},
{48, "EFORMAT", "unformatted or incompatible media"},
{49, "ENOLCK", "no locks available"},
{50, "ENOCONNECT", "cannot Establish Connection"},
{52, "ESTALE", "missing file or filesystem"},
{53, "EDIST", "requests blocked by Administrator"},
{55, "EINPROGRESS", "operation now in progress"},
{56, "EALREADY", "operation already in progress"},
{57, "ENOTSOCK", "socket operation on non-socket"},
{58, "EDESTADDREQ", "destination address required"},
{59, "EMSGSIZE", "message too long"},
{60, "EPROTOTYPE", "protocol wrong type for socket"},
{61, "ENOPROTOOPT", "protocol not available"},
{62, "EPROTONOSUPPORT", "protocol not supported"},
{63, "ESOCKTNOSUPPORT", "socket type not supported"},
{64, "EOPNOTSUPP", "operation not supported on socket"},
{65, "EPFNOSUPPORT", "protocol family not supported"},
{66, "EAFNOSUPPORT", "addr family not supported by protocol"},
{67, "EADDRINUSE", "address already in use"},
{68, "EADDRNOTAVAIL", "can't assign requested address"},
{69, "ENETDOWN", "network is down"},
{70, "ENETUNREACH", "network is unreachable"},
{71, "ENETRESET", "network dropped connection on reset"},
{72, "ECONNABORTED", "software caused connection abort"},
{73, "ECONNRESET", "connection reset by peer"},
{74, "ENOBUFS", "no buffer space available"},
{75, "EISCONN", "socket is already connected"},
{76, "ENOTCONN", "socket is not connected"},
{77, "ESHUTDOWN", "can't send after socket shutdown"},
{78, "ETIMEDOUT", "connection timed out"},
{79, "ECONNREFUSED", "connection refused"},
{80, "EHOSTDOWN", "host is down"},
{81, "EHOSTUNREACH", "no route to host"},
{82, "ERESTART", "restart the system call"},
{83, "EPROCLIM", "too many processes"},
{84, "EUSERS", "too many users"},
{85, "ELOOP", "too many levels of symbolic links"},
{86, "ENAMETOOLONG", "file name too long"},
{88, "EDQUOT", "disk quota exceeded"},
{89, "ECORRUPT", "invalid file system control data detected"},
{90, "ESYSERROR", "for future use "},
{93, "EREMOTE", "item is not local to host"},
{94, "ENOTRECOVERABLE", "state not recoverable "},
{95, "EOWNERDEAD", "previous owner died "},
{109, "ENOSYS", "function not implemented"},
{110, "EMEDIA", "media surface error"},
{111, "ESOFT", "I/O completed, but needs relocation"},
{112, "ENOATTR", "no attribute found"},
{113, "ESAD", "security Authentication Denied"},
{114, "ENOTRUST", "not a Trusted Program"},
{115, "ETOOMANYREFS", "too many references: can't splice"},
{116, "EILSEQ", "invalid wide character"},
{117, "ECANCELED", "asynchronous I/O cancelled"},
{118, "ENOSR", "out of STREAMS resources"},
{119, "ETIME", "system call timed out"},
{120, "EBADMSG", "next message has wrong type"},
{121, "EPROTO", "error in protocol"},
{122, "ENODATA", "no message on stream head read q"},
{123, "ENOSTR", "fd not associated with a stream"},
{124, "ENOTSUP", "unsupported attribute value"},
{125, "EMULTIHOP", "multihop is not allowed"},
{126, "ENOLINK", "the server link has been severed"},
{127, "EOVERFLOW", "value too large to be stored in data type"},
}
// Signal table
var signalList = [...]struct {
num syscall.Signal
name string
desc string
}{
{1, "SIGHUP", "hangup"},
{2, "SIGINT", "interrupt"},
{3, "SIGQUIT", "quit"},
{4, "SIGILL", "illegal instruction"},
{5, "SIGTRAP", "trace/BPT trap"},
{6, "SIGIOT", "IOT/Abort trap"},
{7, "SIGEMT", "EMT trap"},
{8, "SIGFPE", "floating point exception"},
{9, "SIGKILL", "killed"},
{10, "SIGBUS", "bus error"},
{11, "SIGSEGV", "segmentation fault"},
{12, "SIGSYS", "bad system call"},
{13, "SIGPIPE", "broken pipe"},
{14, "SIGALRM", "alarm clock"},
{15, "SIGTERM", "terminated"},
{16, "SIGURG", "urgent I/O condition"},
{17, "SIGSTOP", "stopped (signal)"},
{18, "SIGTSTP", "stopped"},
{19, "SIGCONT", "continued"},
{20, "SIGCHLD", "child exited"},
{21, "SIGTTIN", "stopped (tty input)"},
{22, "SIGTTOU", "stopped (tty output)"},
{23, "SIGIO", "I/O possible/complete"},
{24, "SIGXCPU", "cputime limit exceeded"},
{25, "SIGXFSZ", "filesize limit exceeded"},
{27, "SIGMSG", "input device data"},
{28, "SIGWINCH", "window size changes"},
{29, "SIGPWR", "power-failure"},
{30, "SIGUSR1", "user defined signal 1"},
{31, "SIGUSR2", "user defined signal 2"},
{32, "SIGPROF", "profiling timer expired"},
{33, "SIGDANGER", "paging space low"},
{34, "SIGVTALRM", "virtual timer expired"},
{35, "SIGMIGRATE", "signal 35"},
{36, "SIGPRE", "signal 36"},
{37, "SIGVIRT", "signal 37"},
{38, "SIGTALRM", "signal 38"},
{39, "SIGWAITING", "signal 39"},
{48, "SIGSYSERROR", "signal 48"},
{49, "SIGCAPI", "signal 49"},
{58, "SIGRECONFIG", "signal 58"},
{59, "SIGCPUFAIL", "CPU Failure Predicted"},
{60, "SIGGRANT", "monitor mode granted"},
{61, "SIGRETRACT", "monitor mode retracted"},
{62, "SIGSOUND", "sound completed"},
{63, "SIGMAX32", "secure attention"},
{255, "SIGMAX", "signal 255"},
}
| {
"pile_set_name": "Github"
} |
//===--- StaticallyConstructedObjectsCheck.h - clang-tidy--------*- C++ -*-===//
//
// Part of the LLVM Project, under the Apache License v2.0 with LLVM Exceptions.
// See https://llvm.org/LICENSE.txt for license information.
// SPDX-License-Identifier: Apache-2.0 WITH LLVM-exception
//
//===----------------------------------------------------------------------===//
#ifndef LLVM_CLANG_TOOLS_EXTRA_CLANG_TIDY_FUCHSIA_STATICALLY_CONSTRUCTED_OBJECTS_H
#define LLVM_CLANG_TOOLS_EXTRA_CLANG_TIDY_FUCHSIA_STATICALLY_CONSTRUCTED_OBJECTS_H
#include "../ClangTidyCheck.h"
namespace clang {
namespace tidy {
namespace fuchsia {
/// Constructing global, non-trivial objects with static storage is
/// disallowed, unless the object is statically initialized with a constexpr
/// constructor or has no explicit constructor.
///
/// For the user-facing documentation see:
/// http://clang.llvm.org/extra/clang-tidy/checks/fuchsia-statically-constructed-objects.html
class StaticallyConstructedObjectsCheck : public ClangTidyCheck {
public:
StaticallyConstructedObjectsCheck(StringRef Name, ClangTidyContext *Context)
: ClangTidyCheck(Name, Context) {}
bool isLanguageVersionSupported(const LangOptions &LangOpts) const override {
return LangOpts.CPlusPlus11;
}
void registerMatchers(ast_matchers::MatchFinder *Finder) override;
void check(const ast_matchers::MatchFinder::MatchResult &Result) override;
};
} // namespace fuchsia
} // namespace tidy
} // namespace clang
#endif // LLVM_CLANG_TOOLS_EXTRA_CLANG_TIDY_FUCHSIA_STATICALLY_CONSTRUCTED_OBJECTS_H
| {
"pile_set_name": "Github"
} |
//
// CenterCell.h
// BanTang
//
// Created by liaoyp on 15/4/22.
// Copyright (c) 2015年 JiuZhouYunDong. All rights reserved.
//
#import "BaseTableViewCell.h"
#import "HorizontalScrollCellDeleagte.h"
@interface HorizontalScrollCell : BaseTableViewCell <UICollectionViewDataSource, UICollectionViewDelegate>
{
}
@property(nonatomic ,strong)UICollectionView *collectionView;
@property (weak, nonatomic) id <HorizontalScrollCellDeleagte> delegate;
///**
// Control the Collection View of all Content Cells in each horizontally scrolled row
// */
//@property (strong, nonatomic) UICollectionView *horizontalScrollContentsView;
//
///**
// Set the content cell Class Name
// */
//@property (weak, nonatomic) NSString *contentCellClass;
//
///**
// Tell the size of Content Cell frame.
// Read-only property.
// */
//@property (nonatomic, readonly) CGSize contentCellSize;
//
///**
// Store the table-view index path where each ASOXScrollTableViewCell object is attached to.
// */
@property (strong, nonatomic) NSIndexPath *tableViewIndexPath;
- (void)reloadData;
@end
| {
"pile_set_name": "Github"
} |
; RUN: not llvm-as < %s -disable-output 2>&1 | FileCheck %s
; CHECK-NOT: error:
!0 = !GenericDINode(tag: 65535)
; CHECK: <stdin>:[[@LINE+1]]:26: error: value for 'tag' too large, limit is 65535
!1 = !GenericDINode(tag: 65536)
| {
"pile_set_name": "Github"
} |
[Unit]
Description=Web2py daemon
After=network.target
[Service]
Type=simple
ExecStart=/var/www/web2py/web2py.py --nogui -a '<recycle>' -i 0.0.0.0 -p 8000
User=www-data
[Install]
WantedBy=multi-user.target
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>CFBundleDevelopmentRegion</key>
<string>en</string>
<key>CFBundleExecutable</key>
<string>$(EXECUTABLE_NAME)</string>
<key>CFBundleIdentifier</key>
<string>$(PRODUCT_BUNDLE_IDENTIFIER)</string>
<key>CFBundleInfoDictionaryVersion</key>
<string>6.0</string>
<key>CFBundleName</key>
<string>$(PRODUCT_NAME)</string>
<key>CFBundlePackageType</key>
<string>APPL</string>
<key>CFBundleShortVersionString</key>
<string>1.0</string>
<key>CFBundleSignature</key>
<string>????</string>
<key>CFBundleVersion</key>
<string>1</string>
<key>LSRequiresIPhoneOS</key>
<true/>
<key>UILaunchStoryboardName</key>
<string>LaunchScreen</string>
<key>UIMainStoryboardFile</key>
<string>Main</string>
<key>UIRequiredDeviceCapabilities</key>
<array>
<string>armv7</string>
</array>
<key>UISupportedInterfaceOrientations</key>
<array>
<string>UIInterfaceOrientationPortrait</string>
<string>UIInterfaceOrientationLandscapeLeft</string>
<string>UIInterfaceOrientationLandscapeRight</string>
</array>
<key>UISupportedInterfaceOrientations~ipad</key>
<array>
<string>UIInterfaceOrientationPortrait</string>
<string>UIInterfaceOrientationPortraitUpsideDown</string>
<string>UIInterfaceOrientationLandscapeLeft</string>
<string>UIInterfaceOrientationLandscapeRight</string>
</array>
</dict>
</plist>
| {
"pile_set_name": "Github"
} |
/* This file is part of PyMesh. Copyright (c) 2015 by Qingnan Zhou */
#include "ElementWiseIsotropicMaterial.h"
#include <cassert>
#include <sstream>
#include <Core/Exception.h>
#include "Material.h"
using namespace PyMesh;
ElementWiseIsotropicMaterial::ElementWiseIsotropicMaterial(
Float density, MeshPtr material_mesh,
const std::string& young_field_name,
const std::string& poisson_field_name) :
ElementWiseMaterial(density, material_mesh),
m_young_field_name(young_field_name),
m_poisson_field_name(poisson_field_name) {
if (!m_material_mesh->has_attribute(m_young_field_name)) {
std::stringstream err_msg;
err_msg << "Cannot find attribute: " << m_young_field_name;
throw RuntimeError(err_msg.str());
}
if (!m_material_mesh->has_attribute(m_poisson_field_name)) {
std::stringstream err_msg;
err_msg << "Cannot find attribute: " << m_young_field_name;
throw RuntimeError(err_msg.str());
}
update();
}
void ElementWiseIsotropicMaterial::update() {
const size_t dim = m_material_mesh->get_dim();
if (dim == 2) {
update_2D();
} else if (dim == 3) {
update_3D();
} else {
std::stringstream err_msg;
err_msg << "Unknow dimention: " << dim;
throw NotImplementedError(err_msg.str());
}
}
void ElementWiseIsotropicMaterial::update_2D() {
const size_t num_faces = m_material_mesh->get_num_faces();
const VectorF& young = m_material_mesh->get_attribute(m_young_field_name);
const VectorF& poisson = m_material_mesh->get_attribute(m_poisson_field_name);
assert(young.size() == num_faces);
assert(poisson.size() == num_faces);
m_materials.resize(num_faces);
for (size_t i=0; i<num_faces; i++) {
m_materials[i] = Material::create_isotropic(2, m_density,
young[i], poisson[i]);
}
}
void ElementWiseIsotropicMaterial::update_3D() {
const size_t num_voxels = m_material_mesh->get_num_voxels();
const VectorF& young = m_material_mesh->get_attribute(m_young_field_name);
const VectorF& poisson = m_material_mesh->get_attribute(m_poisson_field_name);
assert(young.size() == num_voxels);
assert(poisson.size() == num_voxels);
m_materials.resize(num_voxels);
for (size_t i=0; i<num_voxels; i++) {
m_materials[i] = Material::create_isotropic(3, m_density,
young[i], poisson[i]);
}
}
| {
"pile_set_name": "Github"
} |
%s:4 PhanTypeMismatchArgumentNullableInternal Argument 2 ($cmp_function) is $closure of type ?\Closure but \usort() takes callable(mixed,mixed):int (expected type to be non-nullable)
| {
"pile_set_name": "Github"
} |
//
// echoprint-codegen
// Copyright 2011 The Echo Nest Corporation. All rights reserved.
//
#ifndef CODEGEN_H
#define CODEGEN_H
// Entry point for generating codes from PCM data.
#define ECHOPRINT_VERSION 4.12
#include <string>
#include <vector>
#ifdef _MSC_VER
#ifdef CODEGEN_EXPORTS
#define CODEGEN_API __declspec(dllexport)
#pragma message("Exporting codegen.dll")
#else
#define CODEGEN_API __declspec(dllimport)
#pragma message("Importing codegen.dll")
#endif
#else
#define CODEGEN_API
#endif
class Fingerprint;
class SubbandAnalysis;
struct FPCode;
class CODEGEN_API Codegen {
public:
Codegen(const float* pcm, unsigned int numSamples, int start_offset);
std::string getCodeString(){return _CodeString;}
int getNumCodes(){return _NumCodes;}
static double getVersion() { return ECHOPRINT_VERSION; }
private:
Fingerprint* computeFingerprint(SubbandAnalysis *pSubbandAnalysis, int start_offset);
std::string createCodeString(std::vector<FPCode> vCodes);
std::string compress(const std::string& s);
std::string _CodeString;
int _NumCodes;
};
#endif
| {
"pile_set_name": "Github"
} |
StartFontMetrics 2.0
Comment Generated by pfaedit
Comment Creation Date: Fri Nov 22 10:56:43 2002
FontName NimbusSanL-ReguCond
FullName Nimbus Sans L Regular Condensed
FamilyName Nimbus Sans L
Weight Regular
Notice (Copyright (URW)++,Copyright 1999 by (URW)++ Design & Development; Cyrillic glyphs added by Valek Filippov (C) 2001-2002)
ItalicAngle 0
IsFixedPitch false
UnderlinePosition -100
UnderlineThickness 50
Version 1.06
EncodingScheme AdobeStandardEncoding
FontBBox -136 -282 893 959
CapHeight 718
XHeight 523
Ascender 718
Descender -207
StartCharMetrics 558
C 0 ; WX 228 ; N .notdef ; B 0 0 0 0 ;
C 32 ; WX 228 ; N space ; B 0 0 0 0 ;
C 33 ; WX 228 ; N exclam ; B 74 0 153 718 ;
C 34 ; WX 291 ; N quotedbl ; B 57 463 234 718 ;
C 35 ; WX 456 ; N numbersign ; B 23 0 434 688 ;
C 36 ; WX 456 ; N dollar ; B 26 -115 427 775 ;
C 37 ; WX 729 ; N percent ; B 32 -19 697 703 ;
C 38 ; WX 547 ; N ampersand ; B 36 -15 529 718 ;
C 39 ; WX 182 ; N quoteright ; B 43 462 129 718 ;
C 40 ; WX 273 ; N parenleft ; B 56 -207 245 733 ;
C 41 ; WX 273 ; N parenright ; B 28 -207 217 733 ;
C 42 ; WX 319 ; N asterisk ; B 32 431 286 718 ;
C 43 ; WX 479 ; N plus ; B 32 0 447 505 ;
C 44 ; WX 228 ; N comma ; B 71 -147 157 107 ;
C 45 ; WX 273 ; N hyphen ; B 36 232 237 322 ;
C 46 ; WX 228 ; N period ; B 71 0 157 107 ;
C 47 ; WX 228 ; N slash ; B -14 -19 242 737 ;
C 48 ; WX 456 ; N zero ; B 30 -19 426 703 ;
C 49 ; WX 456 ; N one ; B 83 0 294 703 ;
C 50 ; WX 456 ; N two ; B 21 0 416 703 ;
C 51 ; WX 456 ; N three ; B 28 -19 428 703 ;
C 52 ; WX 456 ; N four ; B 20 0 429 703 ;
C 53 ; WX 456 ; N five ; B 26 -19 421 688 ;
C 54 ; WX 456 ; N six ; B 31 -19 425 703 ;
C 55 ; WX 456 ; N seven ; B 30 0 429 688 ;
C 56 ; WX 456 ; N eight ; B 31 -19 424 703 ;
C 57 ; WX 456 ; N nine ; B 34 -19 421 703 ;
C 58 ; WX 228 ; N colon ; B 71 0 157 516 ;
C 59 ; WX 228 ; N semicolon ; B 71 -147 157 516 ;
C 60 ; WX 479 ; N less ; B 39 10 440 496 ;
C 61 ; WX 479 ; N equal ; B 32 115 447 390 ;
C 62 ; WX 479 ; N greater ; B 39 10 440 496 ;
C 63 ; WX 456 ; N question ; B 46 0 403 727 ;
C 64 ; WX 832 ; N at ; B 121 -19 712 737 ;
C 65 ; WX 547 ; N A ; B 11 0 536 718 ;
C 66 ; WX 547 ; N B ; B 61 0 514 718 ;
C 67 ; WX 592 ; N C ; B 36 -19 558 737 ;
C 68 ; WX 592 ; N D ; B 66 0 553 718 ;
C 69 ; WX 547 ; N E ; B 71 0 505 718 ;
C 70 ; WX 501 ; N F ; B 71 0 478 718 ;
C 71 ; WX 638 ; N G ; B 39 -19 577 737 ;
C 72 ; WX 592 ; N H ; B 63 0 530 718 ;
C 73 ; WX 228 ; N I ; B 75 0 154 718 ; L J IJ ;
C 74 ; WX 410 ; N J ; B 14 -19 351 718 ;
C 75 ; WX 547 ; N K ; B 62 0 544 718 ;
C 76 ; WX 456 ; N L ; B 62 0 440 718 ;
C 77 ; WX 683 ; N M ; B 60 0 624 718 ;
C 78 ; WX 592 ; N N ; B 62 0 530 718 ;
C 79 ; WX 638 ; N O ; B 32 -19 606 737 ;
C 80 ; WX 547 ; N P ; B 71 0 510 718 ;
C 81 ; WX 638 ; N Q ; B 32 -56 606 737 ;
C 82 ; WX 592 ; N R ; B 72 0 561 718 ;
C 83 ; WX 547 ; N S ; B 40 -19 508 737 ;
C 84 ; WX 501 ; N T ; B 11 0 490 718 ;
C 85 ; WX 592 ; N U ; B 65 -19 528 718 ;
C 86 ; WX 547 ; N V ; B 16 0 531 718 ;
C 87 ; WX 774 ; N W ; B 13 0 761 718 ;
C 88 ; WX 547 ; N X ; B 16 0 531 718 ;
C 89 ; WX 547 ; N Y ; B 11 0 535 718 ;
C 90 ; WX 501 ; N Z ; B 19 0 482 718 ;
C 91 ; WX 228 ; N bracketleft ; B 52 -196 205 722 ;
C 92 ; WX 228 ; N backslash ; B -14 -19 242 737 ;
C 93 ; WX 228 ; N bracketright ; B 23 -196 176 722 ;
C 94 ; WX 385 ; N asciicircum ; B -11 264 396 688 ;
C 95 ; WX 456 ; N underscore ; B 0 -125 456 -75 ;
C 96 ; WX 182 ; N quoteleft ; B 53 469 139 725 ;
C 97 ; WX 456 ; N a ; B 30 -15 435 538 ;
C 98 ; WX 456 ; N b ; B 48 -15 424 718 ;
C 99 ; WX 410 ; N c ; B 25 -15 391 538 ;
C 100 ; WX 456 ; N d ; B 29 -15 409 718 ;
C 101 ; WX 456 ; N e ; B 33 -15 423 538 ;
C 102 ; WX 228 ; N f ; B 11 0 215 728 ; L l fl ; L i fi ;
C 103 ; WX 456 ; N g ; B 33 -220 409 538 ;
C 104 ; WX 456 ; N h ; B 53 0 403 718 ;
C 105 ; WX 182 ; N i ; B 55 0 127 718 ; L j ij ;
C 106 ; WX 182 ; N j ; B -13 -210 127 718 ;
C 107 ; WX 410 ; N k ; B 55 0 411 718 ;
C 108 ; WX 182 ; N l ; B 55 0 127 718 ;
C 109 ; WX 683 ; N m ; B 53 0 631 538 ;
C 110 ; WX 456 ; N n ; B 53 0 403 538 ;
C 111 ; WX 456 ; N o ; B 29 -14 427 538 ;
C 112 ; WX 456 ; N p ; B 48 -207 424 538 ;
C 113 ; WX 456 ; N q ; B 29 -207 405 538 ;
C 114 ; WX 273 ; N r ; B 63 0 272 538 ;
C 115 ; WX 410 ; N s ; B 26 -15 380 538 ;
C 116 ; WX 228 ; N t ; B 11 -7 211 669 ;
C 117 ; WX 456 ; N u ; B 56 -15 401 523 ;
C 118 ; WX 410 ; N v ; B 7 0 403 523 ;
C 119 ; WX 592 ; N w ; B 11 0 581 523 ;
C 120 ; WX 410 ; N x ; B 9 0 402 523 ;
C 121 ; WX 410 ; N y ; B 9 -214 401 523 ;
C 122 ; WX 410 ; N z ; B 25 0 385 523 ;
C 123 ; WX 274 ; N braceleft ; B 34 -196 239 722 ;
C 124 ; WX 213 ; N bar ; B 77 -19 137 737 ;
C 125 ; WX 274 ; N braceright ; B 34 -196 239 722 ;
C 126 ; WX 479 ; N asciitilde ; B 50 181 429 322 ;
C 161 ; WX 273 ; N exclamdown ; B 97 -195 176 523 ;
C 162 ; WX 456 ; N cent ; B 42 -115 421 623 ;
C 163 ; WX 456 ; N sterling ; B 27 -16 442 718 ;
C 164 ; WX 137 ; N fraction ; B -136 -19 273 703 ;
C 165 ; WX 456 ; N yen ; B 2 0 453 688 ;
C 166 ; WX 456 ; N florin ; B -9 -207 411 737 ;
C 167 ; WX 456 ; N section ; B 35 -191 420 737 ;
C 168 ; WX 456 ; N currency ; B 23 99 433 603 ;
C 169 ; WX 157 ; N quotesingle ; B 48 463 108 718 ;
C 170 ; WX 273 ; N quotedblleft ; B 31 469 252 725 ;
C 171 ; WX 456 ; N guillemotleft ; B 80 108 376 446 ;
C 172 ; WX 273 ; N guilsinglleft ; B 72 108 201 446 ;
C 173 ; WX 273 ; N guilsinglright ; B 72 108 201 446 ;
C 174 ; WX 410 ; N fi ; B 11 0 356 728 ;
C 175 ; WX 410 ; N fl ; B 11 0 354 728 ;
C 177 ; WX 456 ; N endash ; B 0 240 456 313 ;
C 178 ; WX 456 ; N dagger ; B 35 -159 421 718 ;
C 179 ; WX 456 ; N daggerdbl ; B 35 -159 421 718 ;
C 180 ; WX 228 ; N periodcentered ; B 63 190 166 315 ;
C 182 ; WX 440 ; N paragraph ; B 15 -173 408 718 ;
C 183 ; WX 287 ; N bullet ; B 15 202 273 517 ;
C 184 ; WX 182 ; N quotesinglbase ; B 43 -149 129 107 ;
C 185 ; WX 273 ; N quotedblbase ; B 21 -149 242 107 ;
C 186 ; WX 273 ; N quotedblright ; B 21 462 242 718 ;
C 187 ; WX 456 ; N guillemotright ; B 80 108 376 446 ;
C 188 ; WX 820 ; N ellipsis ; B 94 0 726 107 ;
C 189 ; WX 820 ; N perthousand ; B 6 -19 815 703 ;
C 191 ; WX 501 ; N questiondown ; B 75 -201 432 525 ;
C 193 ; WX 273 ; N grave ; B 11 593 173 734 ;
C 194 ; WX 273 ; N acute ; B 100 593 262 734 ;
C 195 ; WX 273 ; N circumflex ; B 17 593 256 734 ;
C 196 ; WX 273 ; N tilde ; B -3 606 276 722 ;
C 197 ; WX 273 ; N macron ; B 8 627 265 684 ;
C 198 ; WX 273 ; N breve ; B 11 595 263 731 ;
C 199 ; WX 273 ; N dotaccent ; B 99 604 174 706 ;
C 200 ; WX 273 ; N dieresis ; B 33 604 240 706 ;
C 202 ; WX 273 ; N ring ; B 61 572 212 756 ;
C 203 ; WX 273 ; N cedilla ; B 37 -225 212 0 ;
C 205 ; WX 273 ; N hungarumlaut ; B 25 593 335 734 ;
C 206 ; WX 273 ; N ogonek ; B 60 -225 235 0 ;
C 207 ; WX 273 ; N caron ; B 17 593 256 734 ;
C 208 ; WX 820 ; N emdash ; B 0 240 820 313 ;
C 225 ; WX 820 ; N AE ; B 7 0 780 718 ;
C 227 ; WX 303 ; N ordfeminine ; B 20 304 284 737 ;
C 232 ; WX 456 ; N Lslash ; B -16 0 440 718 ;
C 233 ; WX 638 ; N Oslash ; B 32 -19 607 737 ;
C 234 ; WX 820 ; N OE ; B 30 -19 791 737 ;
C 235 ; WX 299 ; N ordmasculine ; B 20 304 280 737 ;
C 241 ; WX 729 ; N ae ; B 30 -15 695 538 ;
C 245 ; WX 228 ; N dotlessi ; B 78 0 150 523 ;
C 248 ; WX 182 ; N lslash ; B -16 0 198 718 ;
C 249 ; WX 501 ; N oslash ; B 23 -22 440 545 ;
C 250 ; WX 774 ; N oe ; B 29 -15 740 538 ;
C 251 ; WX 501 ; N germandbls ; B 55 -15 468 728 ;
C -1 ; WX 547 ; N Adieresis ; B 11 0 536 901 ;
C -1 ; WX 547 ; N Aacute ; B 11 0 536 929 ;
C -1 ; WX 547 ; N Agrave ; B 11 0 536 929 ;
C -1 ; WX 547 ; N Acircumflex ; B 11 0 536 929 ;
C -1 ; WX 547 ; N Abreve ; B 11 0 536 926 ;
C -1 ; WX 547 ; N Atilde ; B 11 0 536 917 ;
C -1 ; WX 547 ; N Aring ; B 11 0 536 944 ;
C -1 ; WX 547 ; N Aogonek ; B 11 -225 567 718 ;
C -1 ; WX 592 ; N Ccedilla ; B 36 -225 558 737 ;
C -1 ; WX 592 ; N Cacute ; B 36 -19 558 903 ;
C -1 ; WX 592 ; N Ccaron ; B 36 -19 558 929 ;
C -1 ; WX 592 ; N Dcaron ; B 66 0 553 929 ;
C -1 ; WX 547 ; N Edieresis ; B 71 0 505 901 ;
C -1 ; WX 547 ; N Eacute ; B 71 0 505 929 ;
C -1 ; WX 547 ; N Egrave ; B 71 0 505 929 ;
C -1 ; WX 547 ; N Ecircumflex ; B 71 0 505 929 ;
C -1 ; WX 547 ; N Ecaron ; B 71 0 505 929 ;
C -1 ; WX 547 ; N Edotaccent ; B 71 0 505 901 ;
C -1 ; WX 547 ; N Eogonek ; B 71 -225 536 718 ;
C -1 ; WX 638 ; N Gbreve ; B 39 -19 577 926 ;
C -1 ; WX 228 ; N Idieresis ; B 11 0 218 901 ;
C -1 ; WX 228 ; N Iacute ; B 75 0 240 903 ;
C -1 ; WX 228 ; N Igrave ; B -11 0 154 929 ;
C -1 ; WX 228 ; N Icircumflex ; B -5 0 234 929 ;
C -1 ; WX 228 ; N Idotaccent ; B 75 0 154 901 ;
C -1 ; WX 456 ; N Lacute ; B 62 0 440 903 ;
C -1 ; WX 456 ; N Lcaron ; B 62 0 440 718 ;
C -1 ; WX 592 ; N Nacute ; B 62 0 530 903 ;
C -1 ; WX 592 ; N Ncaron ; B 62 0 530 929 ;
C -1 ; WX 592 ; N Ntilde ; B 62 0 530 917 ;
C -1 ; WX 638 ; N Odieresis ; B 32 -19 606 901 ;
C -1 ; WX 638 ; N Oacute ; B 32 -19 606 929 ;
C -1 ; WX 638 ; N Ograve ; B 32 -19 606 929 ;
C -1 ; WX 638 ; N Ocircumflex ; B 32 -19 606 929 ;
C -1 ; WX 638 ; N Otilde ; B 32 -19 606 917 ;
C -1 ; WX 638 ; N Ohungarumlaut ; B 32 -19 606 929 ;
C -1 ; WX 592 ; N Racute ; B 72 0 561 903 ;
C -1 ; WX 592 ; N Rcaron ; B 72 0 561 929 ;
C -1 ; WX 547 ; N Sacute ; B 40 -19 508 903 ;
C -1 ; WX 547 ; N Scaron ; B 40 -19 508 929 ;
C -1 ; WX 547 ; N Scedilla ; B 39 -225 508 737 ;
C -1 ; WX 501 ; N Tcaron ; B 11 0 490 929 ;
C -1 ; WX 592 ; N Udieresis ; B 65 -19 528 901 ;
C -1 ; WX 592 ; N Uacute ; B 65 -19 528 929 ;
C -1 ; WX 592 ; N Ugrave ; B 65 -19 528 929 ;
C -1 ; WX 592 ; N Ucircumflex ; B 65 -19 528 929 ;
C -1 ; WX 592 ; N Uring ; B 65 -19 528 951 ;
C -1 ; WX 592 ; N Uhungarumlaut ; B 65 -19 528 929 ;
C -1 ; WX 547 ; N Yacute ; B 11 0 535 929 ;
C -1 ; WX 501 ; N Zacute ; B 19 0 482 903 ;
C -1 ; WX 501 ; N Zcaron ; B 19 0 482 929 ;
C -1 ; WX 501 ; N Zdotaccent ; B 19 0 482 901 ;
C -1 ; WX 547 ; N Amacron ; B 11 0 536 879 ;
C -1 ; WX 501 ; N Tcommaaccent ; B 11 -282 490 718 ;
C -1 ; WX 547 ; N Ydieresis ; B 11 0 535 901 ;
C -1 ; WX 547 ; N Emacron ; B 71 0 505 879 ;
C -1 ; WX 228 ; N Imacron ; B -15 0 242 879 ;
C -1 ; WX 228 ; N Iogonek ; B 10 -225 185 718 ;
C -1 ; WX 547 ; N Kcommaaccent ; B 62 -282 544 718 ;
C -1 ; WX 456 ; N Lcommaaccent ; B 62 -282 440 718 ;
C -1 ; WX 592 ; N Ncommaaccent ; B 62 -282 530 718 ;
C -1 ; WX 638 ; N Omacron ; B 32 -19 606 879 ;
C -1 ; WX 592 ; N Rcommaaccent ; B 72 -282 561 718 ;
C -1 ; WX 638 ; N Gcommaaccent ; B 39 -282 577 737 ;
C -1 ; WX 592 ; N Umacron ; B 65 -19 528 879 ;
C -1 ; WX 592 ; N Uogonek ; B 65 -225 528 718 ;
C -1 ; WX 456 ; N adieresis ; B 30 -15 435 706 ;
C -1 ; WX 456 ; N aacute ; B 30 -15 435 734 ;
C -1 ; WX 456 ; N agrave ; B 30 -15 435 734 ;
C -1 ; WX 456 ; N acircumflex ; B 30 -15 435 734 ;
C -1 ; WX 456 ; N abreve ; B 30 -15 435 731 ;
C -1 ; WX 456 ; N atilde ; B 30 -15 435 722 ;
C -1 ; WX 456 ; N aring ; B 30 -15 435 769 ;
C -1 ; WX 456 ; N aogonek ; B 30 -225 466 538 ;
C -1 ; WX 410 ; N cacute ; B 25 -15 391 734 ;
C -1 ; WX 410 ; N ccaron ; B 25 -15 391 734 ;
C -1 ; WX 410 ; N ccedilla ; B 25 -225 391 538 ;
C -1 ; WX 496 ; N dcaron ; B 29 -15 516 718 ;
C -1 ; WX 456 ; N edieresis ; B 33 -15 423 706 ;
C -1 ; WX 456 ; N eacute ; B 33 -15 423 734 ;
C -1 ; WX 456 ; N egrave ; B 33 -15 423 734 ;
C -1 ; WX 456 ; N ecircumflex ; B 33 -15 423 734 ;
C -1 ; WX 456 ; N ecaron ; B 33 -15 423 734 ;
C -1 ; WX 456 ; N edotaccent ; B 33 -15 423 706 ;
C -1 ; WX 456 ; N eogonek ; B 33 -225 423 538 ;
C -1 ; WX 456 ; N gbreve ; B 33 -220 409 731 ;
C -1 ; WX 228 ; N idieresis ; B 11 0 218 706 ;
C -1 ; WX 228 ; N iacute ; B 78 0 240 734 ;
C -1 ; WX 228 ; N igrave ; B -11 0 151 734 ;
C -1 ; WX 228 ; N icircumflex ; B -5 0 234 734 ;
C -1 ; WX 182 ; N lacute ; B 55 0 217 903 ;
C -1 ; WX 212 ; N lcaron ; B 55 0 232 718 ;
C -1 ; WX 456 ; N nacute ; B 53 0 403 734 ;
C -1 ; WX 456 ; N ncaron ; B 53 0 403 734 ;
C -1 ; WX 456 ; N ntilde ; B 53 0 403 722 ;
C -1 ; WX 456 ; N odieresis ; B 29 -14 427 706 ;
C -1 ; WX 456 ; N oacute ; B 29 -14 427 734 ;
C -1 ; WX 456 ; N ograve ; B 29 -14 427 734 ;
C -1 ; WX 456 ; N ocircumflex ; B 29 -14 427 734 ;
C -1 ; WX 456 ; N otilde ; B 29 -14 427 722 ;
C -1 ; WX 456 ; N ohungarumlaut ; B 29 -14 427 734 ;
C -1 ; WX 273 ; N racute ; B 63 0 272 734 ;
C -1 ; WX 410 ; N sacute ; B 26 -15 380 734 ;
C -1 ; WX 410 ; N scaron ; B 26 -15 380 734 ;
C -1 ; WX 410 ; N scommaaccent ; B 26 -282 380 538 ;
C -1 ; WX 248 ; N tcaron ; B 11 -7 268 718 ;
C -1 ; WX 456 ; N udieresis ; B 56 -15 401 706 ;
C -1 ; WX 456 ; N uacute ; B 56 -15 401 734 ;
C -1 ; WX 456 ; N ugrave ; B 56 -15 401 734 ;
C -1 ; WX 456 ; N ucircumflex ; B 56 -15 401 734 ;
C -1 ; WX 456 ; N uring ; B 56 -15 401 756 ;
C -1 ; WX 456 ; N uhungarumlaut ; B 56 -15 427 734 ;
C -1 ; WX 410 ; N yacute ; B 9 -214 401 734 ;
C -1 ; WX 410 ; N zacute ; B 25 0 385 734 ;
C -1 ; WX 410 ; N zcaron ; B 25 0 385 734 ;
C -1 ; WX 410 ; N zdotaccent ; B 25 0 385 706 ;
C -1 ; WX 410 ; N ydieresis ; B 9 -214 401 706 ;
C -1 ; WX 228 ; N tcommaaccent ; B 11 -282 211 669 ;
C -1 ; WX 456 ; N amacron ; B 30 -15 435 684 ;
C -1 ; WX 456 ; N emacron ; B 33 -15 423 684 ;
C -1 ; WX 228 ; N imacron ; B -15 0 242 684 ;
C -1 ; WX 410 ; N kcommaaccent ; B 55 -282 411 718 ;
C -1 ; WX 182 ; N lcommaaccent ; B 50 -282 133 718 ;
C -1 ; WX 456 ; N ncommaaccent ; B 53 -282 403 538 ;
C -1 ; WX 456 ; N omacron ; B 29 -14 427 684 ;
C -1 ; WX 273 ; N rcommaaccent ; B 57 -282 272 538 ;
C -1 ; WX 456 ; N umacron ; B 56 -15 401 684 ;
C -1 ; WX 456 ; N uogonek ; B 56 -225 432 523 ;
C -1 ; WX 273 ; N rcaron ; B 44 0 283 734 ;
C -1 ; WX 410 ; N scedilla ; B 26 -225 380 538 ;
C -1 ; WX 456 ; N gcommaaccent ; B 33 -220 409 813 ;
C -1 ; WX 182 ; N iogonek ; B -17 -225 158 718 ;
C -1 ; WX 547 ; N Scommaaccent ; B 40 -282 508 737 ;
C -1 ; WX 592 ; N Eth ; B 0 0 553 718 ;
C -1 ; WX 592 ; N Dcroat ; B 0 0 553 718 ;
C -1 ; WX 547 ; N Thorn ; B 71 0 510 718 ;
C -1 ; WX 456 ; N dcroat ; B 29 -15 456 718 ;
C -1 ; WX 456 ; N eth ; B 29 -15 428 737 ;
C -1 ; WX 456 ; N thorn ; B 48 -207 424 718 ;
C -1 ; WX 510 ; N Euro ; B 0 -12 495 730 ;
C -1 ; WX 273 ; N onesuperior ; B 35 281 182 703 ;
C -1 ; WX 273 ; N twosuperior ; B 3 280 265 714 ;
C -1 ; WX 273 ; N threesuperior ; B 4 270 266 714 ;
C -1 ; WX 328 ; N degree ; B 44 411 284 703 ;
C -1 ; WX 479 ; N minus ; B 32 216 447 289 ;
C -1 ; WX 479 ; N multiply ; B 32 0 447 506 ;
C -1 ; WX 479 ; N divide ; B 32 -19 447 524 ;
C -1 ; WX 820 ; N trademark ; B 38 306 740 718 ;
C -1 ; WX 479 ; N plusminus ; B 32 0 447 561 ;
C -1 ; WX 684 ; N onehalf ; B 35 -19 634 703 ;
C -1 ; WX 684 ; N onequarter ; B 60 -19 620 703 ;
C -1 ; WX 684 ; N threequarters ; B 37 -19 664 714 ;
C -1 ; WX 273 ; N commaaccent ; B 95 -282 178 -60 ;
C -1 ; WX 604 ; N copyright ; B -11 -19 617 737 ;
C -1 ; WX 604 ; N registered ; B -11 -19 617 737 ;
C -1 ; WX 405 ; N lozenge ; B 15 0 382 740 ;
C -1 ; WX 502 ; N Delta ; B 5 0 499 688 ;
C -1 ; WX 479 ; N notequal ; B 32 10 447 495 ;
C -1 ; WX 450 ; N radical ; B -5 -74 433 927 ;
C -1 ; WX 479 ; N lessequal ; B 39 0 439 594 ;
C -1 ; WX 479 ; N greaterequal ; B 39 0 439 594 ;
C -1 ; WX 479 ; N logicalnot ; B 32 108 447 390 ;
C -1 ; WX 585 ; N summation ; B 12 -123 570 752 ;
C -1 ; WX 405 ; N partialdiff ; B 21 -10 379 753 ;
C -1 ; WX 213 ; N brokenbar ; B 77 -19 137 737 ;
C -1 ; WX 456 ; N mu ; B 56 -207 401 523 ;
C -1 ; WX 547 ; N afii10017 ; B 11 0 536 718 ;
C -1 ; WX 551 ; N afii10018 ; B 61 0 514 718 ;
C -1 ; WX 547 ; N afii10019 ; B 61 0 514 718 ;
C -1 ; WX 489 ; N afii10020 ; B 61 0 468 718 ;
C -1 ; WX 666 ; N afii10021 ; B 21 -120 645 718 ;
C -1 ; WX 537 ; N afii10022 ; B 61 0 495 718 ;
C -1 ; WX 537 ; N afii10023 ; B 61 0 495 901 ;
C -1 ; WX 902 ; N afii10024 ; B 9 0 893 718 ;
C -1 ; WX 541 ; N afii10025 ; B 42 -19 510 737 ;
C -1 ; WX 596 ; N afii10026 ; B 61 0 529 718 ;
C -1 ; WX 596 ; N afii10027 ; B 61 0 529 878 ;
C -1 ; WX 552 ; N afii10028 ; B 61 0 543 718 ;
C -1 ; WX 581 ; N afii10029 ; B 21 -10 514 718 ;
C -1 ; WX 692 ; N afii10030 ; B 61 0 625 718 ;
C -1 ; WX 595 ; N afii10031 ; B 61 0 528 718 ;
C -1 ; WX 638 ; N afii10032 ; B 32 -19 606 737 ;
C -1 ; WX 595 ; N afii10033 ; B 61 0 528 718 ;
C -1 ; WX 547 ; N afii10034 ; B 61 0 500 718 ;
C -1 ; WX 588 ; N afii10035 ; B 32 -19 554 737 ;
C -1 ; WX 503 ; N afii10036 ; B 12 0 491 718 ;
C -1 ; WX 548 ; N afii10037 ; B 12 0 536 718 ;
C -1 ; WX 737 ; N afii10038 ; B 34 0 703 718 ;
C -1 ; WX 539 ; N afii10039 ; B 12 0 527 718 ;
C -1 ; WX 627 ; N afii10040 ; B 61 -120 599 718 ;
C -1 ; WX 567 ; N afii10041 ; B 62 0 500 718 ;
C -1 ; WX 698 ; N afii10042 ; B 61 0 631 718 ;
C -1 ; WX 723 ; N afii10043 ; B 61 -120 702 718 ;
C -1 ; WX 711 ; N afii10044 ; B 12 0 674 718 ;
C -1 ; WX 723 ; N afii10045 ; B 61 0 656 718 ;
C -1 ; WX 537 ; N afii10046 ; B 61 0 500 718 ;
C -1 ; WX 590 ; N afii10047 ; B 32 -19 554 737 ;
C -1 ; WX 813 ; N afii10048 ; B 61 -19 781 737 ;
C -1 ; WX 554 ; N afii10049 ; B 42 0 487 718 ;
C -1 ; WX 456 ; N afii10065 ; B 30 -15 435 538 ;
C -1 ; WX 442 ; N afii10066 ; B 25 -14 424 776 ;
C -1 ; WX 444 ; N afii10067 ; B 54 0 420 524 ;
C -1 ; WX 337 ; N afii10068 ; B 51 0 325 523 ;
C -1 ; WX 536 ; N afii10069 ; B 21 -125 515 523 ;
C -1 ; WX 456 ; N afii10070 ; B 33 -15 423 538 ;
C -1 ; WX 456 ; N afii10071 ; B 33 -15 423 706 ;
C -1 ; WX 656 ; N afii10072 ; B 8 0 646 523 ;
C -1 ; WX 410 ; N afii10073 ; B 24 -15 378 538 ;
C -1 ; WX 455 ; N afii10074 ; B 53 0 403 524 ;
C -1 ; WX 455 ; N afii10075 ; B 53 0 403 739 ;
C -1 ; WX 417 ; N afii10076 ; B 53 0 409 523 ;
C -1 ; WX 439 ; N afii10077 ; B 21 -10 387 523 ;
C -1 ; WX 539 ; N afii10078 ; B 53 0 487 523 ;
C -1 ; WX 455 ; N afii10079 ; B 53 0 403 525 ;
C -1 ; WX 456 ; N afii10080 ; B 29 -14 427 538 ;
C -1 ; WX 455 ; N afii10081 ; B 53 0 403 523 ;
C -1 ; WX 456 ; N afii10082 ; B 48 -207 424 538 ;
C -1 ; WX 410 ; N afii10083 ; B 25 -15 391 538 ;
C -1 ; WX 336 ; N afii10084 ; B 12 0 324 523 ;
C -1 ; WX 409 ; N afii10085 ; B 8 -214 400 523 ;
C -1 ; WX 748 ; N afii10086 ; B 32 -207 716 628 ;
C -1 ; WX 410 ; N afii10087 ; B 8 0 401 523 ;
C -1 ; WX 474 ; N afii10088 ; B 53 -125 455 523 ;
C -1 ; WX 443 ; N afii10089 ; B 53 0 391 525 ;
C -1 ; WX 589 ; N afii10090 ; B 53 0 537 523 ;
C -1 ; WX 606 ; N afii10091 ; B 53 -125 589 523 ;
C -1 ; WX 512 ; N afii10092 ; B 17 0 497 524 ;
C -1 ; WX 585 ; N afii10093 ; B 53 0 533 524 ;
C -1 ; WX 444 ; N afii10094 ; B 53 0 419 524 ;
C -1 ; WX 410 ; N afii10095 ; B 25 -15 391 538 ;
C -1 ; WX 597 ; N afii10096 ; B 53 -14 568 538 ;
C -1 ; WX 447 ; N afii10097 ; B 24 0 410 524 ;
C -1 ; WX 537 ; N uni0400 ; B 61 0 495 959 ;
C -1 ; WX 688 ; N afii10051 ; B 12 0 651 718 ;
C -1 ; WX 489 ; N afii10052 ; B 61 0 468 919 ;
C -1 ; WX 588 ; N afii10053 ; B 32 -19 554 737 ;
C -1 ; WX 547 ; N afii10054 ; B 40 -19 508 737 ;
C -1 ; WX 213 ; N afii10055 ; B 67 0 146 718 ;
C -1 ; WX 341 ; N afii10056 ; B 67 0 274 901 ;
C -1 ; WX 410 ; N afii10057 ; B 14 -19 351 718 ;
C -1 ; WX 581 ; N afii10058 ; B 21 0 514 718 ;
C -1 ; WX 924 ; N afii10059 ; B 61 0 887 718 ;
C -1 ; WX 622 ; N afii10060 ; B 12 0 569 718 ;
C -1 ; WX 552 ; N afii10061 ; B 61 0 543 919 ;
C -1 ; WX 596 ; N uni040D ; B 61 0 529 959 ;
C -1 ; WX 546 ; N afii10062 ; B 11 0 535 878 ;
C -1 ; WX 595 ; N afii10145 ; B 61 0 528 718 ;
C -1 ; WX 456 ; N uni0450 ; B 33 -15 423 764 ;
C -1 ; WX 526 ; N afii10099 ; B 12 0 501 523 ;
C -1 ; WX 337 ; N afii10100 ; B 51 0 325 748 ;
C -1 ; WX 426 ; N afii10101 ; B 29 -15 395 538 ;
C -1 ; WX 410 ; N afii10102 ; B 26 -15 380 538 ;
C -1 ; WX 182 ; N afii10103 ; B 55 0 127 718 ;
C -1 ; WX 317 ; N afii10104 ; B 55 0 262 706 ;
C -1 ; WX 182 ; N afii10105 ; B -13 -210 127 718 ;
C -1 ; WX 439 ; N afii10106 ; B 21 0 387 523 ;
C -1 ; WX 722 ; N afii10107 ; B 53 0 697 525 ;
C -1 ; WX 535 ; N afii10108 ; B 12 0 482 718 ;
C -1 ; WX 417 ; N afii10109 ; B 53 0 409 748 ;
C -1 ; WX 455 ; N uni045D ; B 53 0 403 764 ;
C -1 ; WX 416 ; N afii10110 ; B 12 -214 404 741 ;
C -1 ; WX 455 ; N afii10193 ; B 53 0 403 523 ;
C -1 ; WX 537 ; N uni048C ; B 61 0 500 718 ;
C -1 ; WX 444 ; N uni048D ; B 53 0 419 524 ;
C -1 ; WX 547 ; N uni048E ; B 61 0 500 718 ;
C -1 ; WX 456 ; N uni048F ; B 48 -207 424 538 ;
C -1 ; WX 499 ; N afii10050 ; B 67 0 474 799 ;
C -1 ; WX 344 ; N afii10098 ; B 55 0 329 591 ;
C -1 ; WX 489 ; N uni0492 ; B 61 0 468 718 ;
C -1 ; WX 337 ; N uni0493 ; B 51 0 325 523 ;
C -1 ; WX 489 ; N uni0494 ; B 61 0 468 718 ;
C -1 ; WX 337 ; N uni0495 ; B 51 0 325 523 ;
C -1 ; WX 902 ; N uni0496 ; B 9 0 893 718 ;
C -1 ; WX 658 ; N uni0497 ; B 8 0 650 523 ;
C -1 ; WX 541 ; N uni0498 ; B 42 -19 510 737 ;
C -1 ; WX 410 ; N uni0499 ; B 24 -15 378 538 ;
C -1 ; WX 552 ; N uni049A ; B 61 0 543 718 ;
C -1 ; WX 417 ; N uni049B ; B 53 0 409 523 ;
C -1 ; WX 552 ; N uni049C ; B 61 0 543 718 ;
C -1 ; WX 417 ; N uni049D ; B 53 0 409 523 ;
C -1 ; WX 552 ; N uni049E ; B 61 0 543 718 ;
C -1 ; WX 417 ; N uni049F ; B 53 0 409 523 ;
C -1 ; WX 552 ; N uni04A0 ; B 61 0 543 718 ;
C -1 ; WX 417 ; N uni04A1 ; B 53 0 409 523 ;
C -1 ; WX 595 ; N uni04A2 ; B 61 0 528 718 ;
C -1 ; WX 455 ; N uni04A3 ; B 53 0 403 525 ;
C -1 ; WX 595 ; N uni04A4 ; B 61 0 528 718 ;
C -1 ; WX 455 ; N uni04A5 ; B 53 0 403 525 ;
C -1 ; WX 595 ; N uni04A6 ; B 61 0 528 718 ;
C -1 ; WX 455 ; N uni04A7 ; B 53 0 403 523 ;
C -1 ; WX 588 ; N uni04A8 ; B 32 -19 554 737 ;
C -1 ; WX 410 ; N uni04A9 ; B 25 -15 391 538 ;
C -1 ; WX 588 ; N uni04AA ; B 32 -19 554 737 ;
C -1 ; WX 410 ; N uni04AB ; B 25 -15 391 538 ;
C -1 ; WX 503 ; N uni04AC ; B 12 0 491 718 ;
C -1 ; WX 336 ; N uni04AD ; B 12 0 324 523 ;
C -1 ; WX 547 ; N uni04AE ; B 11 0 535 718 ;
C -1 ; WX 410 ; N uni04AF ; B 7 0 403 523 ;
C -1 ; WX 547 ; N uni04B0 ; B 11 0 535 718 ;
C -1 ; WX 410 ; N uni04B1 ; B 7 0 403 523 ;
C -1 ; WX 539 ; N uni04B2 ; B 12 0 527 718 ;
C -1 ; WX 410 ; N uni04B3 ; B 8 0 401 523 ;
C -1 ; WX 645 ; N uni04B4 ; B 61 -120 624 718 ;
C -1 ; WX 501 ; N uni04B5 ; B 53 -125 480 523 ;
C -1 ; WX 547 ; N uni04B6 ; B 62 0 480 718 ;
C -1 ; WX 406 ; N uni04B7 ; B 52 0 371 525 ;
C -1 ; WX 547 ; N uni04B8 ; B 62 0 480 718 ;
C -1 ; WX 406 ; N uni04B9 ; B 52 0 371 525 ;
C -1 ; WX 547 ; N uni04BA ; B 62 0 480 718 ;
C -1 ; WX 406 ; N uni04BB ; B 53 0 372 525 ;
C -1 ; WX 588 ; N uni04BC ; B 32 -19 554 737 ;
C -1 ; WX 410 ; N uni04BD ; B 25 -15 391 538 ;
C -1 ; WX 588 ; N uni04BE ; B 32 -19 554 737 ;
C -1 ; WX 410 ; N uni04BF ; B 25 -15 391 538 ;
C -1 ; WX 228 ; N uni04C0 ; B 75 0 154 718 ;
C -1 ; WX 902 ; N uni04C1 ; B 9 0 893 954 ;
C -1 ; WX 658 ; N uni04C2 ; B 8 0 650 759 ;
C -1 ; WX 552 ; N uni04C3 ; B 61 0 543 718 ;
C -1 ; WX 417 ; N uni04C4 ; B 53 0 409 523 ;
C -1 ; WX 595 ; N uni04C7 ; B 61 0 528 718 ;
C -1 ; WX 455 ; N uni04C8 ; B 53 0 403 525 ;
C -1 ; WX 547 ; N uni04CB ; B 62 0 480 718 ;
C -1 ; WX 406 ; N uni04CC ; B 52 0 371 525 ;
C -1 ; WX 547 ; N uni04D0 ; B 11 0 536 954 ;
C -1 ; WX 456 ; N uni04D1 ; B 30 -15 435 759 ;
C -1 ; WX 547 ; N uni04D2 ; B 11 0 536 920 ;
C -1 ; WX 456 ; N uni04D3 ; B 30 -15 435 725 ;
C -1 ; WX 820 ; N uni04D4 ; B 7 0 780 718 ;
C -1 ; WX 729 ; N uni04D5 ; B 30 -15 695 538 ;
C -1 ; WX 537 ; N uni04D6 ; B 61 0 495 954 ;
C -1 ; WX 456 ; N uni04D7 ; B 33 -15 423 759 ;
C -1 ; WX 588 ; N uni04D8 ; B 32 -19 554 737 ;
C -1 ; WX 410 ; N afii10846 ; B 25 -15 391 538 ;
C -1 ; WX 588 ; N uni04DA ; B 32 -19 554 920 ;
C -1 ; WX 410 ; N uni04DB ; B 25 -15 391 725 ;
C -1 ; WX 902 ; N uni04DC ; B 9 0 893 920 ;
C -1 ; WX 658 ; N uni04DD ; B 8 0 650 725 ;
C -1 ; WX 541 ; N uni04DE ; B 42 -19 510 920 ;
C -1 ; WX 410 ; N uni04DF ; B 24 -15 378 725 ;
C -1 ; WX 541 ; N uni04E0 ; B 42 -19 510 737 ;
C -1 ; WX 410 ; N uni04E1 ; B 24 -15 378 538 ;
C -1 ; WX 596 ; N uni04E2 ; B 61 0 529 875 ;
C -1 ; WX 455 ; N uni04E3 ; B 53 0 403 680 ;
C -1 ; WX 596 ; N uni04E4 ; B 61 0 529 920 ;
C -1 ; WX 455 ; N uni04E5 ; B 53 0 403 725 ;
C -1 ; WX 638 ; N uni04E6 ; B 32 -19 606 920 ;
C -1 ; WX 456 ; N uni04E7 ; B 29 -14 427 725 ;
C -1 ; WX 638 ; N uni04E8 ; B 32 -19 606 737 ;
C -1 ; WX 456 ; N uni04E9 ; B 29 -14 427 538 ;
C -1 ; WX 638 ; N uni04EA ; B 32 -19 606 920 ;
C -1 ; WX 456 ; N uni04EB ; B 29 -14 427 725 ;
C -1 ; WX 590 ; N uni04EC ; B 32 -19 554 920 ;
C -1 ; WX 410 ; N uni04ED ; B 25 -15 391 725 ;
C -1 ; WX 548 ; N uni04EE ; B 12 0 536 875 ;
C -1 ; WX 409 ; N uni04EF ; B 8 -214 400 680 ;
C -1 ; WX 548 ; N uni04F0 ; B 12 0 536 920 ;
C -1 ; WX 409 ; N uni04F1 ; B 8 -214 400 725 ;
C -1 ; WX 548 ; N uni04F2 ; B 12 0 536 959 ;
C -1 ; WX 409 ; N uni04F3 ; B 8 -214 400 764 ;
C -1 ; WX 547 ; N uni04F4 ; B 62 0 480 920 ;
C -1 ; WX 406 ; N uni04F5 ; B 52 0 371 725 ;
C -1 ; WX 783 ; N uni04F8 ; B 61 0 716 920 ;
C -1 ; WX 585 ; N uni04F9 ; B 53 0 533 725 ;
C -1 ; WX 592 ; N Ccircumflex ; B 36 -19 558 959 ;
C -1 ; WX 410 ; N ccircumflex ; B 25 -15 391 764 ;
C -1 ; WX 592 ; N Cdotaccent ; B 36 -19 558 920 ;
C -1 ; WX 410 ; N cdotaccent ; B 25 -15 391 725 ;
C -1 ; WX 547 ; N Ebreve ; B 71 0 505 954 ;
C -1 ; WX 456 ; N ebreve ; B 33 -15 423 759 ;
C -1 ; WX 638 ; N Gcircumflex ; B 39 -19 577 959 ;
C -1 ; WX 456 ; N gcircumflex ; B 33 -220 409 764 ;
C -1 ; WX 638 ; N Gdotaccent ; B 39 -19 577 920 ;
C -1 ; WX 456 ; N gdotaccent ; B 33 -220 409 725 ;
C -1 ; WX 592 ; N Hcircumflex ; B 63 0 530 959 ;
C -1 ; WX 456 ; N hcircumflex ; B -30 0 403 959 ;
C -1 ; WX 592 ; N Hbar ; B 63 0 530 718 ;
C -1 ; WX 456 ; N hbar ; B 53 0 403 718 ;
C -1 ; WX 228 ; N Itilde ; B -25 0 254 934 ;
C -1 ; WX 228 ; N itilde ; B -26 0 253 739 ;
C -1 ; WX 228 ; N Ibreve ; B -12 0 240 954 ;
C -1 ; WX 228 ; N ibreve ; B -12 0 240 759 ;
C -1 ; WX 644 ; N IJ ; B 75 -19 566 718 ;
C -1 ; WX 308 ; N ij ; B 55 -210 254 718 ;
C -1 ; WX 410 ; N Jcircumflex ; B 14 -19 431 959 ;
C -1 ; WX 182 ; N jcircumflex ; B -27 -210 211 786 ;
C -1 ; WX 417 ; N kgreenlandic ; B 53 0 409 523 ;
C -1 ; WX 456 ; N Ldot ; B 62 0 440 718 ;
C -1 ; WX 410 ; N ldot ; B 55 0 348 718 ;
C -1 ; WX 456 ; N napostrophe ; B 53 0 403 538 ;
C -1 ; WX 592 ; N Eng ; B 62 0 530 718 ;
C -1 ; WX 456 ; N eng ; B 53 0 403 538 ;
C -1 ; WX 638 ; N Obreve ; B 32 -19 606 954 ;
C -1 ; WX 456 ; N obreve ; B 29 -14 427 759 ;
C -1 ; WX 547 ; N Scircumflex ; B 40 -19 508 959 ;
C -1 ; WX 410 ; N scircumflex ; B 26 -15 380 764 ;
C -1 ; WX 501 ; N uni0162 ; B 11 -225 490 718 ;
C -1 ; WX 228 ; N uni0163 ; B 11 -232 227 669 ;
C -1 ; WX 501 ; N Tbar ; B 11 0 490 718 ;
C -1 ; WX 228 ; N tbar ; B 11 -7 211 669 ;
C -1 ; WX 592 ; N Utilde ; B 65 -19 528 934 ;
C -1 ; WX 456 ; N utilde ; B 56 -15 401 739 ;
C -1 ; WX 592 ; N Ubreve ; B 65 -19 528 954 ;
C -1 ; WX 456 ; N ubreve ; B 56 -15 401 759 ;
C -1 ; WX 774 ; N Wcircumflex ; B 13 0 761 959 ;
C -1 ; WX 592 ; N wcircumflex ; B 11 0 581 764 ;
C -1 ; WX 547 ; N Ycircumflex ; B 11 0 535 959 ;
C -1 ; WX 410 ; N ycircumflex ; B 9 -214 401 764 ;
C -1 ; WX 228 ; N longs ; B 11 0 215 728 ;
EndCharMetrics
StartKernData
StartKernPairs 978
KPX quoteright A -60
KPX quoteright AE -63
KPX quoteright Aacute -60
KPX quoteright Adieresis -60
KPX quoteright Aring -60
KPX quoteright comma -48
KPX quoteright d -16
KPX quoteright o -24
KPX quoteright period -48
KPX quoteright r -15
KPX quoteright s -13
KPX quoteright t -3
KPX quoteright w 1
KPX comma one -83
KPX comma quotedblright -22
KPX comma quoteright -33
KPX hyphen A -3
KPX hyphen AE -4
KPX hyphen Aacute -3
KPX hyphen Adieresis -3
KPX hyphen Aring -3
KPX hyphen T -61
KPX hyphen V -29
KPX hyphen W -9
KPX hyphen Y -67
KPX period one -83
KPX period quotedblright -22
KPX period quoteright -33
KPX zero four 5
KPX zero one -31
KPX zero seven -21
KPX one comma -54
KPX one eight -46
KPX one five -49
KPX one four -59
KPX one nine -47
KPX one one -90
KPX one period -54
KPX one seven -64
KPX one six -44
KPX one three -51
KPX one two -50
KPX one zero -43
KPX two four -38
KPX two one -29
KPX two seven -14
KPX three four 8
KPX three one -34
KPX three seven -15
KPX four four 8
KPX four one -65
KPX four seven -39
KPX five four 4
KPX five one -56
KPX five seven -16
KPX six four 6
KPX six one -31
KPX six seven -13
KPX seven colon -47
KPX seven comma -95
KPX seven eight -15
KPX seven five -22
KPX seven four -72
KPX seven one -34
KPX seven period -95
KPX seven seven 3
KPX seven six -24
KPX seven three -14
KPX seven two -14
KPX eight four 6
KPX eight one -36
KPX eight seven -16
KPX nine four 1
KPX nine one -31
KPX nine seven -19
KPX A C -28
KPX A Ccedilla -29
KPX A G -30
KPX A O -27
KPX A Odieresis -27
KPX A Q -28
KPX A T -74
KPX A U -29
KPX A Uacute -29
KPX A Ucircumflex -29
KPX A Udieresis -29
KPX A Ugrave -29
KPX A V -56
KPX A W -39
KPX A Y -78
KPX A a -3
KPX A c -10
KPX A ccedilla -10
KPX A comma 5
KPX A d -11
KPX A e -14
KPX A g -14
KPX A guillemotleft -40
KPX A guilsinglleft -36
KPX A hyphen -2
KPX A o -13
KPX A period 5
KPX A q -11
KPX A quotedblright -37
KPX A quoteright -48
KPX A t -15
KPX A u -12
KPX A v -27
KPX A w -21
KPX A y -27
KPX B A -15
KPX B AE -14
KPX B Aacute -15
KPX B Acircumflex -15
KPX B Adieresis -15
KPX B Aring -15
KPX B Atilde -15
KPX B O -3
KPX B Oacute -3
KPX B Ocircumflex -3
KPX B Odieresis -3
KPX B Ograve -3
KPX B V -25
KPX B W -14
KPX B Y -31
KPX C A -25
KPX C AE -24
KPX C Aacute -25
KPX C Adieresis -25
KPX C Aring -25
KPX C H -6
KPX C K -5
KPX C O -4
KPX C Oacute -4
KPX C Odieresis -4
KPX D A -33
KPX D Aacute -33
KPX D Acircumflex -33
KPX D Adieresis -33
KPX D Agrave -33
KPX D Aring -33
KPX D Atilde -33
KPX D J -1
KPX D T -30
KPX D V -32
KPX D W -18
KPX D X -38
KPX D Y -44
KPX F A -55
KPX F Aacute -55
KPX F Acircumflex -55
KPX F Adieresis -55
KPX F Agrave -55
KPX F Aring -55
KPX F Atilde -55
KPX F J -50
KPX F O -15
KPX F Odieresis -15
KPX F a -26
KPX F aacute -26
KPX F adieresis -26
KPX F ae -26
KPX F aring -26
KPX F comma -102
KPX F e -19
KPX F eacute -19
KPX F hyphen -12
KPX F i -8
KPX F j -8
KPX F o -17
KPX F oacute -17
KPX F odieresis -17
KPX F oe -17
KPX F oslash -17
KPX F period -102
KPX F r -32
KPX F u -28
KPX G A -4
KPX G AE -2
KPX G Aacute -4
KPX G Acircumflex -4
KPX G Adieresis -4
KPX G Agrave -4
KPX G Aring -4
KPX G Atilde -4
KPX G T -30
KPX G V -36
KPX G W -20
KPX G Y -47
KPX J A -22
KPX J AE -21
KPX J Adieresis -22
KPX J Aring -22
KPX K C -37
KPX K G -40
KPX K O -37
KPX K OE -33
KPX K Oacute -37
KPX K Odieresis -37
KPX K S -27
KPX K T 22
KPX K a -6
KPX K adieresis -6
KPX K ae -7
KPX K aring -6
KPX K e -26
KPX K hyphen -38
KPX K o -26
KPX K oacute -26
KPX K odieresis -26
KPX K u -21
KPX K udieresis -21
KPX K y -52
KPX L A 18
KPX L AE 20
KPX L Aacute 18
KPX L Adieresis 18
KPX L Aring 18
KPX L C -28
KPX L Ccedilla -32
KPX L G -31
KPX L O -29
KPX L Oacute -29
KPX L Ocircumflex -29
KPX L Odieresis -29
KPX L Ograve -29
KPX L Otilde -29
KPX L S -11
KPX L T -81
KPX L U -25
KPX L Udieresis -25
KPX L V -78
KPX L W -50
KPX L Y -92
KPX L hyphen -110
KPX L quotedblright -105
KPX L quoteright -116
KPX L u -9
KPX L udieresis -9
KPX L y -47
KPX N A -4
KPX N AE -2
KPX N Aacute -4
KPX N Adieresis -4
KPX N Aring -4
KPX N G -1
KPX N O 1
KPX N Oacute 1
KPX N Odieresis 1
KPX N a -1
KPX N aacute -1
KPX N adieresis -1
KPX N ae -1
KPX N aring -1
KPX N comma -4
KPX N e 1
KPX N eacute 1
KPX N o 1
KPX N oacute 1
KPX N odieresis 1
KPX N oslash 4
KPX N period -4
KPX O A -29
KPX O AE -29
KPX O Aacute -29
KPX O Adieresis -29
KPX O Aring -29
KPX O T -27
KPX O V -30
KPX O W -14
KPX O X -35
KPX O Y -42
KPX P A -62
KPX P AE -64
KPX P Aacute -62
KPX P Adieresis -62
KPX P Aring -62
KPX P J -70
KPX P a -21
KPX P aacute -21
KPX P adieresis -21
KPX P ae -21
KPX P aring -21
KPX P comma -123
KPX P e -24
KPX P eacute -24
KPX P hyphen -28
KPX P o -24
KPX P oacute -24
KPX P odieresis -24
KPX P oe -22
KPX P oslash -22
KPX P period -123
KPX R C -7
KPX R Ccedilla -7
KPX R G -9
KPX R O -6
KPX R OE -3
KPX R Oacute -6
KPX R Odieresis -6
KPX R T -12
KPX R U -8
KPX R Udieresis -8
KPX R V -22
KPX R W -15
KPX R Y -29
KPX R a -6
KPX R aacute -6
KPX R adieresis -6
KPX R ae -6
KPX R aring -6
KPX R e -5
KPX R eacute -5
KPX R hyphen 4
KPX R o -5
KPX R oacute -5
KPX R odieresis -5
KPX R oe -5
KPX R u -4
KPX R uacute -5
KPX R udieresis -5
KPX R y -1
KPX S A -15
KPX S AE -14
KPX S Aacute -15
KPX S Adieresis -15
KPX S Aring -15
KPX S T -14
KPX S V -25
KPX S W -17
KPX S Y -31
KPX S t -2
KPX T A -78
KPX T AE -76
KPX T Aacute -78
KPX T Acircumflex -78
KPX T Adieresis -78
KPX T Agrave -78
KPX T Aring -78
KPX T Atilde -78
KPX T C -27
KPX T G -31
KPX T J -80
KPX T O -26
KPX T OE -22
KPX T Oacute -26
KPX T Ocircumflex -26
KPX T Odieresis -26
KPX T Ograve -26
KPX T Oslash -27
KPX T Otilde -26
KPX T S -15
KPX T V 17
KPX T W 19
KPX T Y 19
KPX T a -79
KPX T ae -79
KPX T c -73
KPX T colon -95
KPX T comma -80
KPX T e -77
KPX T g -76
KPX T guillemotleft -100
KPX T guilsinglleft -96
KPX T hyphen -60
KPX T i -2
KPX T j -2
KPX T o -76
KPX T oslash -72
KPX T period -80
KPX T r -77
KPX T s -74
KPX T semicolon -93
KPX T u -75
KPX T v -79
KPX T w -80
KPX T y -79
KPX U A -32
KPX U AE -32
KPX U Aacute -32
KPX U Acircumflex -32
KPX U Adieresis -32
KPX U Aring -32
KPX U Atilde -32
KPX U comma -24
KPX U m -1
KPX U n -1
KPX U period -22
KPX U r -6
KPX V A -58
KPX V AE -60
KPX V Aacute -58
KPX V Acircumflex -58
KPX V Adieresis -58
KPX V Agrave -58
KPX V Aring -58
KPX V Atilde -58
KPX V C -31
KPX V G -34
KPX V O -30
KPX V Oacute -30
KPX V Ocircumflex -30
KPX V Odieresis -30
KPX V Ograve -30
KPX V Oslash -27
KPX V Otilde -30
KPX V S -26
KPX V T 18
KPX V a -47
KPX V ae -47
KPX V colon -41
KPX V comma -73
KPX V e -46
KPX V g -44
KPX V guillemotleft -68
KPX V guilsinglleft -64
KPX V hyphen -29
KPX V i -5
KPX V o -46
KPX V oslash -41
KPX V period -73
KPX V r -37
KPX V semicolon -41
KPX V u -35
KPX V y -12
KPX W A -42
KPX W AE -43
KPX W Aacute -42
KPX W Acircumflex -42
KPX W Adieresis -42
KPX W Agrave -42
KPX W Aring -42
KPX W Atilde -42
KPX W C -15
KPX W G -18
KPX W O -14
KPX W Oacute -14
KPX W Ocircumflex -14
KPX W Odieresis -14
KPX W Ograve -14
KPX W Oslash -12
KPX W Otilde -14
KPX W S -19
KPX W T 20
KPX W a -29
KPX W ae -29
KPX W colon -31
KPX W comma -46
KPX W e -26
KPX W g -24
KPX W guillemotleft -48
KPX W guilsinglleft -44
KPX W hyphen -9
KPX W i -3
KPX W o -26
KPX W oslash -21
KPX W period -46
KPX W r -26
KPX W semicolon -31
KPX W u -24
KPX W y -2
KPX X C -33
KPX X O -33
KPX X Odieresis -33
KPX X Q -33
KPX X a -12
KPX X e -31
KPX X hyphen -40
KPX X o -31
KPX X u -27
KPX X y -42
KPX Y A -80
KPX Y AE -82
KPX Y Aacute -80
KPX Y Acircumflex -80
KPX Y Adieresis -80
KPX Y Agrave -80
KPX Y Aring -80
KPX Y Atilde -80
KPX Y C -43
KPX Y G -47
KPX Y O -43
KPX Y Oacute -43
KPX Y Ocircumflex -43
KPX Y Odieresis -43
KPX Y Ograve -43
KPX Y Oslash -44
KPX Y Otilde -43
KPX Y S -33
KPX Y T 20
KPX Y a -73
KPX Y ae -73
KPX Y colon -60
KPX Y comma -92
KPX Y e -74
KPX Y g -73
KPX Y guillemotleft -103
KPX Y guilsinglleft -99
KPX Y hyphen -68
KPX Y i -3
KPX Y o -74
KPX Y oslash -69
KPX Y p -48
KPX Y period -92
KPX Y semicolon -60
KPX Y u -54
KPX Y v -31
KPX Z v -24
KPX Z y -25
KPX quoteleft A -52
KPX quoteleft AE -55
KPX quoteleft Aacute -52
KPX quoteleft Adieresis -52
KPX quoteleft Aring -52
KPX quoteleft T 5
KPX quoteleft V 13
KPX quoteleft W 20
KPX quoteleft Y 3
KPX a j -5
KPX a quoteright -10
KPX a v -19
KPX a w -14
KPX a y -20
KPX b v -13
KPX b w -8
KPX b y -15
KPX c h 2
KPX c k 1
KPX e quoteright -5
KPX e t -8
KPX e v -16
KPX e w -11
KPX e x -19
KPX e y -18
KPX f a -9
KPX f aacute -9
KPX f adieresis -9
KPX f ae -9
KPX f aring -9
KPX f e -12
KPX f eacute -12
KPX f f 17
KPX f i -5
KPX f j -5
KPX f l -5
KPX f o -12
KPX f oacute -12
KPX f odieresis -12
KPX f oe -12
KPX f oslash -8
KPX f quoteright 12
KPX f s -4
KPX f t 17
KPX g a 1
KPX g adieresis 1
KPX g ae 1
KPX g aring 1
KPX g e 4
KPX g eacute 4
KPX g l 4
KPX g oacute 4
KPX g odieresis 4
KPX h quoteright -3
KPX h y -14
KPX i T -2
KPX k a -5
KPX k aacute -5
KPX k adieresis -5
KPX k ae -5
KPX k aring -5
KPX k comma 1
KPX k e -19
KPX k eacute -19
KPX k g -18
KPX k hyphen -31
KPX k o -19
KPX k oacute -19
KPX k odieresis -19
KPX k period 1
KPX k s -9
KPX k u -3
KPX k udieresis -3
KPX l v -3
KPX l y -3
KPX m p 4
KPX m v -13
KPX m w -7
KPX m y -13
KPX n T -75
KPX n p 4
KPX n quoteright -3
KPX n v -14
KPX n w -8
KPX n y -14
KPX o T -77
KPX o quoteright -8
KPX o t -7
KPX o v -15
KPX o w -9
KPX o x -18
KPX o y -17
KPX p t -6
KPX p y -15
KPX q c 6
KPX q u 1
KPX r a -3
KPX r aacute -3
KPX r acircumflex -3
KPX r adieresis -3
KPX r ae -3
KPX r agrave -3
KPX r aring -3
KPX r c -6
KPX r ccedilla -3
KPX r colon -5
KPX r comma -48
KPX r d -4
KPX r e -10
KPX r eacute -10
KPX r ecircumflex -10
KPX r egrave -10
KPX r f 23
KPX r g -4
KPX r h 2
KPX r hyphen -30
KPX r i 1
KPX r k 1
KPX r l 1
KPX r m 2
KPX r n 2
KPX r o -11
KPX r oacute -11
KPX r ocircumflex -11
KPX r odieresis -11
KPX r oe -7
KPX r ograve -11
KPX r oslash -7
KPX r p 4
KPX r period -48
KPX r q -4
KPX r quoteright 14
KPX r r -3
KPX r s 2
KPX r semicolon -5
KPX r t 23
KPX r v 24
KPX r w 22
KPX r x 19
KPX r y 23
KPX r z 6
KPX s quoteright -5
KPX s t -5
KPX t S -8
KPX t a 1
KPX t aacute 1
KPX t adieresis 1
KPX t ae 1
KPX t aring 1
KPX t colon -13
KPX t e -10
KPX t eacute -10
KPX t h 1
KPX t o -10
KPX t oacute -10
KPX t odieresis -10
KPX t quoteright 10
KPX t semicolon -13
KPX u quoteright 5
KPX v a -16
KPX v aacute -16
KPX v acircumflex -16
KPX v adieresis -16
KPX v ae -16
KPX v agrave -16
KPX v aring -16
KPX v atilde -16
KPX v c -12
KPX v colon -8
KPX v comma -50
KPX v e -16
KPX v eacute -16
KPX v ecircumflex -16
KPX v egrave -16
KPX v g -15
KPX v hyphen -3
KPX v l -2
KPX v o -16
KPX v oacute -16
KPX v odieresis -16
KPX v ograve -16
KPX v oslash -12
KPX v period -50
KPX v s -10
KPX v semicolon -8
KPX w a -13
KPX w aacute -13
KPX w acircumflex -13
KPX w adieresis -13
KPX w ae -13
KPX w agrave -13
KPX w aring -13
KPX w atilde -13
KPX w c -5
KPX w colon -10
KPX w comma -37
KPX w e -9
KPX w eacute -9
KPX w ecircumflex -9
KPX w egrave -9
KPX w g -8
KPX w hyphen 3
KPX w l -4
KPX w o -9
KPX w oacute -9
KPX w odieresis -9
KPX w ograve -9
KPX w oslash -5
KPX w period -37
KPX w s -7
KPX w semicolon -10
KPX x a -10
KPX x c -13
KPX x e -17
KPX x eacute -17
KPX x o -17
KPX x q -14
KPX y a -16
KPX y aacute -16
KPX y acircumflex -16
KPX y adieresis -16
KPX y ae -16
KPX y agrave -16
KPX y aring -16
KPX y atilde -16
KPX y c -13
KPX y colon -9
KPX y comma -49
KPX y e -17
KPX y eacute -17
KPX y ecircumflex -17
KPX y egrave -17
KPX y g -15
KPX y hyphen -2
KPX y l -3
KPX y o -16
KPX y oacute -16
KPX y odieresis -16
KPX y ograve -16
KPX y oslash -12
KPX y period -49
KPX y s -11
KPX y semicolon -9
KPX quotedblleft A -41
KPX quotedblleft AE -44
KPX quotedblleft Aacute -41
KPX quotedblleft Adieresis -41
KPX quotedblleft Aring -41
KPX quotedblleft T 16
KPX quotedblleft V 24
KPX quotedblleft W 31
KPX quotedblleft Y 14
KPX guilsinglright A -38
KPX guilsinglright AE -39
KPX guilsinglright Aacute -38
KPX guilsinglright Adieresis -38
KPX guilsinglright Aring -38
KPX guilsinglright T -96
KPX guilsinglright V -64
KPX guilsinglright W -43
KPX guilsinglright Y -98
KPX quotedblbase A 24
KPX quotedblbase AE 25
KPX quotedblbase T -60
KPX quotedblbase V -53
KPX quotedblbase W -25
KPX quotedblbase Y -71
KPX quotedblright A -49
KPX quotedblright AE -52
KPX quotedblright Aacute -49
KPX quotedblright Adieresis -49
KPX quotedblright Aring -49
KPX quotedblright T 11
KPX quotedblright V 16
KPX quotedblright W 23
KPX quotedblright Y 9
KPX guillemotright A -42
KPX guillemotright AE -43
KPX guillemotright Aacute -42
KPX guillemotright Adieresis -42
KPX guillemotright Aring -42
KPX guillemotright T -101
KPX guillemotright V -68
KPX guillemotright W -48
KPX guillemotright Y -102
KPX Oslash A -27
KPX ae v -17
KPX ae w -11
KPX ae y -19
KPX Adieresis C -28
KPX Adieresis G -30
KPX Adieresis O -27
KPX Adieresis Q -28
KPX Adieresis T -74
KPX Adieresis U -29
KPX Adieresis V -56
KPX Adieresis W -39
KPX Adieresis Y -78
KPX Adieresis a -3
KPX Adieresis c -10
KPX Adieresis comma 5
KPX Adieresis d -11
KPX Adieresis g -14
KPX Adieresis guillemotleft -40
KPX Adieresis guilsinglleft -36
KPX Adieresis hyphen -2
KPX Adieresis o -13
KPX Adieresis period 5
KPX Adieresis q -11
KPX Adieresis quotedblright -37
KPX Adieresis quoteright -48
KPX Adieresis t -15
KPX Adieresis u -12
KPX Adieresis v -27
KPX Adieresis w -21
KPX Adieresis y -27
KPX Aacute C -28
KPX Aacute G -30
KPX Aacute O -27
KPX Aacute Q -28
KPX Aacute T -74
KPX Aacute U -29
KPX Aacute V -56
KPX Aacute W -39
KPX Aacute Y -78
KPX Aacute a -3
KPX Aacute c -10
KPX Aacute comma 5
KPX Aacute d -11
KPX Aacute e -14
KPX Aacute g -14
KPX Aacute guillemotleft -40
KPX Aacute guilsinglleft -36
KPX Aacute hyphen -2
KPX Aacute o -13
KPX Aacute period 5
KPX Aacute q -11
KPX Aacute quoteright -48
KPX Aacute t -15
KPX Aacute u -12
KPX Aacute v -27
KPX Aacute w -21
KPX Aacute y -27
KPX Agrave C -28
KPX Agrave G -30
KPX Agrave O -27
KPX Agrave Q -28
KPX Agrave T -74
KPX Agrave U -29
KPX Agrave V -56
KPX Agrave W -39
KPX Agrave Y -78
KPX Agrave comma 5
KPX Agrave period 5
KPX Acircumflex C -28
KPX Acircumflex G -30
KPX Acircumflex O -27
KPX Acircumflex Q -28
KPX Acircumflex T -74
KPX Acircumflex U -29
KPX Acircumflex V -56
KPX Acircumflex W -39
KPX Acircumflex Y -78
KPX Acircumflex comma 5
KPX Acircumflex period 5
KPX Atilde C -28
KPX Atilde G -30
KPX Atilde O -27
KPX Atilde Q -28
KPX Atilde T -74
KPX Atilde U -29
KPX Atilde V -56
KPX Atilde W -39
KPX Atilde Y -78
KPX Atilde comma 5
KPX Atilde period 5
KPX Aring C -28
KPX Aring G -30
KPX Aring O -27
KPX Aring Q -28
KPX Aring T -74
KPX Aring U -29
KPX Aring V -56
KPX Aring W -39
KPX Aring Y -78
KPX Aring a -3
KPX Aring c -10
KPX Aring comma 5
KPX Aring d -11
KPX Aring e -14
KPX Aring g -14
KPX Aring guillemotleft -40
KPX Aring guilsinglleft -36
KPX Aring hyphen -2
KPX Aring o -13
KPX Aring period 5
KPX Aring q -11
KPX Aring quotedblright -37
KPX Aring quoteright -48
KPX Aring t -15
KPX Aring u -12
KPX Aring v -27
KPX Aring w -21
KPX Aring y -27
KPX Ccedilla A -28
KPX Odieresis A -29
KPX Odieresis T -27
KPX Odieresis V -30
KPX Odieresis W -14
KPX Odieresis X -35
KPX Odieresis Y -42
KPX Oacute A -29
KPX Oacute T -27
KPX Oacute V -30
KPX Oacute W -14
KPX Oacute Y -42
KPX Ograve T -27
KPX Ograve V -30
KPX Ograve Y -42
KPX Ocircumflex T -27
KPX Ocircumflex V -30
KPX Ocircumflex Y -42
KPX Otilde T -27
KPX Otilde V -30
KPX Otilde Y -42
KPX Udieresis A -32
KPX Udieresis comma -24
KPX Udieresis m -1
KPX Udieresis n -1
KPX Udieresis period -22
KPX Udieresis r -6
KPX Uacute A -32
KPX Uacute comma -24
KPX Uacute m -1
KPX Uacute n -1
KPX Uacute period -22
KPX Uacute r -6
KPX Ugrave A -32
KPX Ucircumflex A -32
KPX adieresis v -19
KPX adieresis w -14
KPX adieresis y -20
KPX aacute v -19
KPX aacute w -14
KPX aacute y -20
KPX agrave v -19
KPX agrave w -14
KPX agrave y -20
KPX aring v -19
KPX aring w -14
KPX aring y -20
KPX eacute v -16
KPX eacute w -11
KPX eacute y -18
KPX ecircumflex v -16
KPX ecircumflex w -11
KPX ecircumflex y -18
KPX odieresis t -7
KPX odieresis v -15
KPX odieresis w -9
KPX odieresis x -18
KPX odieresis y -17
KPX oacute v -15
KPX oacute w -9
KPX oacute y -17
KPX ograve v -15
KPX ograve w -9
KPX ograve y -17
KPX ocircumflex t -7
EndKernPairs
EndKernData
EndFontMetrics
| {
"pile_set_name": "Github"
} |
const EMAIL_PATTERN = /^[A-Za-z0-9\u4e00-\u9fa5]+@[a-zA-Z0-9_-]+(\.[a-zA-Z0-9_-]+)+$/
const ID_CARD_PATTERN = /^(^[1-9]\d{7}((0\d)|(1[0-2]))(([0|1|2]\d)|3[0-1])\d{3}$)|(^[1-9]\d{5}[1-9]\d{3}((0\d)|(1[0-2]))(([0|1|2]\d)|3[0-1])((\d{4})|\d{3}[Xx])$)$/
function doubleCheck (rule, value, callback) {
if (!rule.hasOwnProperty('target')) {
return callback(new Error('请填写 target'))
}
if (rule.target !== value) {
return callback(new Error())
}
callback()
}
function checkPhone (rule, value, cb) {
if (!value) {
return cb(new Error('未填写手机号码'))
} else if (!(/^1(3|4|5|7|8)\d{9}$/.test(value))) {
return cb(new Error('嗨,这不是手机号码'))
} else {
cb()
}
}
function checkEmail (rule, value, cb) {
if (!value) {
return cb(new Error('未填写邮箱'))
} else if (!(EMAIL_PATTERN.test(value))) {
return cb(new Error('嗨,这不是邮箱'))
} else {
cb()
}
}
function checkIdCardNumber (rule, value, cb) {
if (!value) {
return cb(new Error('未填写身份证号码'))
} else if (!(ID_CARD_PATTERN.test(value))) {
return cb(new Error('嗨,身份证号码格式不正确'))
} else {
if (value.length === 18) {
let idCardWi = [7, 9, 10, 5, 8, 4, 2, 1, 6, 3, 7, 9, 10, 5, 8, 4, 2] // 将前17位加权因子保存在数组里
let idCardY = [1, 0, 10, 9, 8, 7, 6, 5, 4, 3, 2] // 这是除以11后,可能产生的11位余数、验证码,也保存成数组
let idCardWiSum = 0 // 用来保存前17位各自乖以加权因子后的总和
for (let i = 0; i < 17; i++) {
idCardWiSum += parseInt(value.substring(i, i + 1)) * idCardWi[i]
}
let idCardMod = idCardWiSum % 11 // 计算出校验码所在数组的位置
let idCardLast = value.substring(17) // 得到最后一位身份证号码
// 如果等于2,则说明校验码是10,身份证号码最后一位应该是X
// 用计算出的验证码与最后一位身份证号码匹配,如果一致,说明通过,否则是无效的身份证号码
if ((idCardMod === 2 && idCardLast.toLowerCase() !== 'x') || (idCardMod !== 2 && parseInt(idCardLast) !== idCardY[idCardMod])) {
return cb(new Error('嗨,身份证号码错误'))
}
} else {
return cb(new Error('嗨,身份证号码错误'))
}
cb()
}
}
export default {
install (Vue) {
Vue.validator = Vue.prototype.$validator = {
doubleCheck,
checkPhone,
checkEmail,
checkIdCardNumber
}
}
}
| {
"pile_set_name": "Github"
} |
set(LLVM_LINK_COMPONENTS support)
set(BUILDVARIABLES_SRCPATH ${CMAKE_CURRENT_SOURCE_DIR}/BuildVariables.inc.in)
set(BUILDVARIABLES_OBJPATH ${CMAKE_CURRENT_BINARY_DIR}/BuildVariables.inc)
# Add the llvm-config tool.
add_llvm_tool(llvm-config
llvm-config.cpp
)
# Compute the substitution values for various items.
get_property(SUPPORT_SYSTEM_LIBS TARGET LLVMSupport PROPERTY LLVM_SYSTEM_LIBS)
get_property(WINDOWSMANIFEST_SYSTEM_LIBS TARGET LLVMWindowsManifest PROPERTY LLVM_SYSTEM_LIBS)
foreach(l ${SUPPORT_SYSTEM_LIBS} ${WINDOWSMANIFEST_SYSTEM_LIBS})
if(MSVC)
set(SYSTEM_LIBS ${SYSTEM_LIBS} "${l}.lib")
else()
if (l MATCHES "^-")
# If it's an option, pass it without changes.
set(SYSTEM_LIBS ${SYSTEM_LIBS} "${l}")
else()
# Otherwise assume it's a library name we need to link with.
set(SYSTEM_LIBS ${SYSTEM_LIBS} "-l${l}")
endif()
endif()
endforeach()
string(REPLACE ";" " " SYSTEM_LIBS "${SYSTEM_LIBS}")
# Fetch target specific compile options, e.g. RTTI option
get_property(COMPILE_FLAGS TARGET llvm-config PROPERTY COMPILE_FLAGS)
# The language standard potentially affects the ABI/API of LLVM, so we want
# to make sure it is reported by llvm-config.
# NOTE: We don't want to start extracting any random C/CXX flags that the
# user may add that could affect the ABI. We only want to extract flags
# that have been added by the LLVM build system.
string(REGEX MATCH "-std=[^ ]\+" LLVM_CXX_STD_FLAG ${CMAKE_CXX_FLAGS})
string(REGEX MATCH "-std=[^ ]\+" LLVM_C_STD_FLAG ${CMAKE_C_FLAGS})
# Use configure_file to create BuildVariables.inc.
set(LLVM_SRC_ROOT ${LLVM_MAIN_SRC_DIR})
set(LLVM_OBJ_ROOT ${LLVM_BINARY_DIR})
set(LLVM_CPPFLAGS "${LLVM_DEFINITIONS}")
set(LLVM_CFLAGS "${LLVM_C_STD_FLAG} ${LLVM_DEFINITIONS}")
set(LLVM_CXXFLAGS "${LLVM_CXX_STD_FLAG} ${COMPILE_FLAGS} ${LLVM_DEFINITIONS}")
set(LLVM_BUILD_SYSTEM cmake)
set(LLVM_HAS_RTTI ${LLVM_CONFIG_HAS_RTTI})
set(LLVM_DYLIB_VERSION "${LLVM_VERSION_MAJOR}${LLVM_VERSION_SUFFIX}")
set(LLVM_HAS_GLOBAL_ISEL "ON")
# Use the C++ link flags, since they should be a superset of C link flags.
set(LLVM_LDFLAGS "${CMAKE_CXX_LINK_FLAGS}")
set(LLVM_BUILDMODE ${CMAKE_BUILD_TYPE})
set(LLVM_SYSTEM_LIBS ${SYSTEM_LIBS})
string(REPLACE ";" " " LLVM_TARGETS_BUILT "${LLVM_TARGETS_TO_BUILD}")
llvm_canonicalize_cmake_booleans(
LLVM_BUILD_LLVM_DYLIB
LLVM_LINK_LLVM_DYLIB
LLVM_HAS_RTTI
LLVM_HAS_GLOBAL_ISEL
BUILD_SHARED_LIBS)
configure_file(${BUILDVARIABLES_SRCPATH} ${BUILDVARIABLES_OBJPATH} @ONLY)
# Set build-time environment(s).
add_definitions(-DCMAKE_CFG_INTDIR="${CMAKE_CFG_INTDIR}")
if(LLVM_ENABLE_MODULES)
target_compile_options(llvm-config PUBLIC
"-fmodules-ignore-macro=CMAKE_CFG_INTDIR"
)
endif()
# Add the dependency on the generation step.
add_file_dependencies(${CMAKE_CURRENT_SOURCE_DIR}/llvm-config.cpp ${BUILDVARIABLES_OBJPATH})
if(CMAKE_CROSSCOMPILING AND NOT LLVM_CONFIG_PATH)
set(LLVM_CONFIG_PATH "${LLVM_NATIVE_BUILD}/bin/llvm-config" CACHE STRING "")
add_custom_command(OUTPUT "${LLVM_CONFIG_PATH}"
COMMAND ${CMAKE_COMMAND} --build . --target llvm-config --config $<CONFIGURATION>
DEPENDS ${LLVM_NATIVE_BUILD}/CMakeCache.txt
WORKING_DIRECTORY ${LLVM_NATIVE_BUILD}
COMMENT "Building native llvm-config..."
USES_TERMINAL)
add_custom_target(NativeLLVMConfig DEPENDS ${LLVM_CONFIG_PATH})
add_dependencies(NativeLLVMConfig CONFIGURE_LLVM_NATIVE)
add_dependencies(llvm-config NativeLLVMConfig)
endif()
| {
"pile_set_name": "Github"
} |
#include <windows.h>
#include <stdio.h>
typedef int (__stdcall *MYPROC) (LPWSTR, LPWSTR, DWORD,LPWSTR, LPDWORD,DWORD);
int main(int argc, char* argv[])
{
WCHAR path[256];
WCHAR can_path[256];
DWORD type = 1000;
int retval;
HMODULE handle = LoadLibrary(".\\netapi32.dll");
MYPROC Trigger = NULL;
if (NULL == handle)
{
wprintf(L"Fail to load library!\n");
return -1;
}
Trigger = (MYPROC)GetProcAddress(handle, "NetpwPathCanonicalize");
if (NULL == Trigger)
{
FreeLibrary(handle);
wprintf(L"Fail to get api address!\n");
return -1;
}
path[0] = 0;
wcscpy(path, L"\\aaa\\..\\..\\bbbb");
can_path[0] = 0;
type = 1000;
wprintf(L"BEFORE: %s\n", path);
retval = (Trigger)(path, can_path, 1000, NULL, &type, 1);
wprintf(L"AFTER : %s\n", can_path);
wprintf(L"RETVAL: %s(0x%X)\n\n", retval?L"FAIL":L"SUCCESS", retval);
FreeLibrary(handle);
return 0;
}
| {
"pile_set_name": "Github"
} |
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.stratos.python.cartridge.agent.integration.tests;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.stratos.common.domain.LoadBalancingIPType;
import org.apache.stratos.messaging.domain.topology.*;
import org.apache.stratos.messaging.event.instance.notifier.ArtifactUpdatedEvent;
import org.apache.stratos.messaging.event.topology.CompleteTopologyEvent;
import org.apache.stratos.messaging.event.topology.MemberInitializedEvent;
import org.testng.annotations.AfterMethod;
import org.testng.annotations.BeforeMethod;
import org.testng.annotations.Test;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
import java.util.Properties;
/**
* To test the agent termination flow by terminator.txt file
*/
public class AgentTerminationTestCase extends PythonAgentIntegrationTest {
public AgentTerminationTestCase() throws IOException {
}
@Override
protected String getClassName() {
return this.getClass().getSimpleName();
}
private static final Log log = LogFactory.getLog(AgentTerminationTestCase.class);
private static final int TIMEOUT = 300000;
private static final String CLUSTER_ID = "tomcat.domain";
private static final String APPLICATION_PATH = "/tmp/AgentTerminationTestCase";
private static final String DEPLOYMENT_POLICY_NAME = "deployment-policy-6";
private static final String AUTOSCALING_POLICY_NAME = "autoscaling-policy-6";
private static final String APP_ID = "application-6";
private static final String MEMBER_ID = "tomcat.member-1";
private static final String INSTANCE_ID = "instance-1";
private static final String CLUSTER_INSTANCE_ID = "cluster-1-instance-1";
private static final String NETWORK_PARTITION_ID = "network-partition-1";
private static final String PARTITION_ID = "partition-1";
private static final String TENANT_ID = "6";
private static final String SERVICE_NAME = "tomcat";
@BeforeMethod(alwaysRun = true)
public void setup() throws Exception {
System.setProperty("jndi.properties.dir", getCommonResourcesPath());
super.setup(TIMEOUT);
startServerSocket(8080);
}
@AfterMethod(alwaysRun = true)
public void tearDownAgentTerminationTest(){
tearDown(APPLICATION_PATH);
}
@Test(groups = {"smoke"})
public void testAgentTerminationByFile() throws IOException {
startCommunicatorThread();
assertAgentActivation();
sleep(5000);
String terminatorFilePath = agentPath + PATH_SEP + "terminator.txt";
log.info("Writing termination flag to " + terminatorFilePath);
File terminatorFile = new File(terminatorFilePath);
String msg = "true";
Files.write(Paths.get(terminatorFile.getAbsolutePath()), msg.getBytes());
log.info("Waiting until agent reads termination flag");
sleep(50000);
List<String> outputLines = new ArrayList<>();
boolean exit = false;
while (!exit) {
List<String> newLines = getNewLines(outputLines, outputStream.toString());
if (newLines.size() > 0) {
for (String line : newLines) {
if (line.contains("Shutting down Stratos cartridge agent...")) {
log.info("Cartridge agent shutdown successfully");
exit = true;
}
}
}
sleep(1000);
}
}
private void assertAgentActivation() {
Thread startupTestThread = new Thread(new Runnable() {
@Override
public void run() {
while (!eventReceiverInitialized) {
sleep(1000);
}
List<String> outputLines = new ArrayList<>();
while (!outputStream.isClosed()) {
List<String> newLines = getNewLines(outputLines, outputStream.toString());
if (newLines.size() > 0) {
for (String line : newLines) {
if (line.contains("Subscribed to 'topology/#'")) {
sleep(2000);
// Send complete topology event
log.info("Publishing complete topology event...");
Topology topology = PythonAgentIntegrationTest.createTestTopology(
SERVICE_NAME,
CLUSTER_ID,
DEPLOYMENT_POLICY_NAME,
AUTOSCALING_POLICY_NAME,
APP_ID,
MEMBER_ID,
CLUSTER_INSTANCE_ID,
NETWORK_PARTITION_ID,
PARTITION_ID,
ServiceType.SingleTenant);
CompleteTopologyEvent completeTopologyEvent = new CompleteTopologyEvent(topology);
publishEvent(completeTopologyEvent);
log.info("Complete topology event published");
// Publish member initialized event
log.info("Publishing member initialized event...");
MemberInitializedEvent memberInitializedEvent = new MemberInitializedEvent(SERVICE_NAME,
CLUSTER_ID, CLUSTER_INSTANCE_ID, MEMBER_ID, NETWORK_PARTITION_ID, PARTITION_ID,
INSTANCE_ID);
publishEvent(memberInitializedEvent);
log.info("Member initialized event published");
}
// Send artifact updated event to activate the instance first
if (line.contains("Artifact repository found")) {
publishEvent(getArtifactUpdatedEventForPrivateRepo());
log.info("Artifact updated event published");
}
}
}
sleep(1000);
}
}
});
startupTestThread.start();
while (!instanceStarted || !instanceActivated) {
// wait until the instance activated event is received.
// this will assert whether instance got activated within timeout period; no need for explicit assertions
sleep(2000);
}
}
public static ArtifactUpdatedEvent getArtifactUpdatedEventForPrivateRepo() {
ArtifactUpdatedEvent privateRepoEvent = createTestArtifactUpdatedEvent();
privateRepoEvent.setRepoURL("https://bitbucket.org/testapache2211/testrepo.git");
privateRepoEvent.setRepoUserName("testapache2211");
privateRepoEvent.setRepoPassword("iF7qT+BKKPE3PGV1TeDsJA==");
return privateRepoEvent;
}
private static ArtifactUpdatedEvent createTestArtifactUpdatedEvent() {
ArtifactUpdatedEvent artifactUpdatedEvent = new ArtifactUpdatedEvent();
artifactUpdatedEvent.setClusterId(CLUSTER_ID);
artifactUpdatedEvent.setTenantId(TENANT_ID);
return artifactUpdatedEvent;
}
}
| {
"pile_set_name": "Github"
} |
<?php
/**
* This file is part of the Carbon package.
*
* (c) Brian Nesbitt <brian@nesbot.com>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
return require __DIR__.'/et.php';
| {
"pile_set_name": "Github"
} |
#ifndef _PART_DRIVER_H
#define _PART_DRIVER_H 1
int part_connect(mass_dev* dev);
void part_disconnect(mass_dev* dev);
#endif
| {
"pile_set_name": "Github"
} |
/* See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* Esri Inc. licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
using System;
using System.Collections.Generic;
using System.Text;
using System.IO;
using System.Net;
namespace com.esri.gpt.csw
{
/// <summary>
/// CswClient class is used to submit CSW search request.
/// </summary>
/// <remarks>
/// CswClient is a wrapper class of .NET HttpWebRequest and HttpWebResponse. It basically submits
/// a HTTP request then return a text response.
/// </remarks>
///
public class CswClient
{
private CredentialCache _credentialCache;
static CookieContainer _cookieContainer; //use static variable to be thread safe
private String downloadFileName = "";
private bool retryAttempt = true;
#region Constructor
/// <summary>
/// Constructor
/// </summary>
public CswClient()
{
_credentialCache = new CredentialCache();
_cookieContainer = new CookieContainer();
}
#endregion
#region PublicMethods
/// <summary>
/// Submit HTTP Request
/// </summary>
/// <param name="method">HTTP Method. for example "POST", "GET"</param>
/// <param name="URL">URL to send HTTP Request to</param>
/// <param name="postdata">Data to be posted</param>
/// <returns>Response in plain text</returns>
public string SubmitHttpRequest(string method, string URL, string postdata)
{
return SubmitHttpRequest(method, URL, postdata, "", "");
}
/// <summary>
/// Submit HTTP Request
/// </summary>
/// <remarks>
/// Submit an HTTP request.
/// </remarks>
/// <param name="method">HTTP Method. for example "POST", "GET"</param>
/// <param name="URL">URL to send HTTP Request to</param>
/// <param name="postdata">Data to be posted</param>
/// <param name="usr">Username</param>
/// <param name="pwd">Password</param>
/// <returns>Response in plain text.</returns>
public string SubmitHttpRequest(string method, string URL, string postdata, string usr, string pwd)
{
String responseText = "";
HttpWebRequest request;
Uri uri = new Uri(URL);
request = (HttpWebRequest)WebRequest.Create(uri);
request.AllowAutoRedirect = true;
if(method.Equals("SOAP")){
request.Method = "POST";
request.Headers.Add("SOAPAction: Some-URI");
request.ContentType = "text/xml; charset=UTF-8";
}
else if (method.Equals("DOWNLOAD"))
{
request.Method = "GET";
}
else{
request.ContentType = "text/xml; charset=UTF-8";
request.Method = method;
}
// Credential and cookies
request.CookieContainer = _cookieContainer;
NetworkCredential nc = null;
String authType = "Negotiate";
if (_credentialCache.GetCredential(uri, authType) == null && _credentialCache.GetCredential(uri, "Basic") == null)
{
if (!String.IsNullOrEmpty(usr) && !String.IsNullOrEmpty(pwd))
{
nc = new NetworkCredential(usr, pwd);
_credentialCache.Add(uri, "Basic", nc);
_credentialCache.Add(uri, authType, nc);
}
else{
nc = System.Net.CredentialCache.DefaultNetworkCredentials;
_credentialCache.Add(uri, authType, nc);
}
}
request.Credentials = _credentialCache;
// post data
if (request.Method.Equals("POST", StringComparison.OrdinalIgnoreCase))
{
UTF8Encoding encoding = new UTF8Encoding();
Byte[] byteTemp = encoding.GetBytes(postdata);
request.ContentLength = byteTemp.Length;
Stream requestStream = request.GetRequestStream();
requestStream.Write(byteTemp, 0, byteTemp.Length);
requestStream.Close();
}
HttpWebResponse response = null;
try{
response = (HttpWebResponse)request.GetResponse();
}
catch (UnauthorizedAccessException ua)
{
if (retryAttempt)
{
PromptCredentials pc = new PromptCredentials();
pc.ShowDialog();
retryAttempt = false;
try
{
_credentialCache.Remove(uri, "Basic");
}
catch (Exception) { };
_credentialCache.Remove(uri, authType);
if (!String.IsNullOrEmpty(pc.Username) && !String.IsNullOrEmpty(pc.Password))
return SubmitHttpRequest(method, URL, postdata, pc.Username, pc.Password);
else
return null;
}
else
{
retryAttempt = true;
throw ua;
}
}
catch (WebException we)
{
if (retryAttempt)
{
PromptCredentials pc = new PromptCredentials();
pc.ShowDialog();
retryAttempt = false;
try
{
_credentialCache.Remove(uri, "Basic");
}catch(Exception){};
_credentialCache.Remove(uri, authType);
if (!String.IsNullOrEmpty(pc.Username) && !String.IsNullOrEmpty(pc.Password))
return SubmitHttpRequest(method, URL, postdata, pc.Username, pc.Password);
else
return null;
}
else
{
retryAttempt = true;
throw we;
}
}
if (_cookieContainer.GetCookies(uri) == null)
{
_cookieContainer.Add(uri, response.Cookies);
}
Stream responseStream = response.GetResponseStream();
if (method.Equals("DOWNLOAD"))
{
FileStream file = null;
string fileName = response.GetResponseHeader("Content-Disposition");
string[] s = null;
if (fileName.ToLower().EndsWith(".tif"))
{
s = URL.Split(new String[] { "coverage=" }, 100, StringSplitOptions.RemoveEmptyEntries);
s[1] = s[1].Trim() + ".tif";
}
else
{
s = fileName.Split('=');
s[1] = s[1].Replace('\\', ' ');
s[1] = s[1].Replace('"', ' ');
s[1] = s[1].Trim();
}
try
{
downloadFileName = System.IO.Path.Combine(Utils.GetSpecialFolderPath(SpecialFolder.ConfigurationFiles), s[1]);
System.IO.File.Delete(downloadFileName);
file = System.IO.File.Create(downloadFileName);
// Buffer to read 10K bytes in chunk:
byte[] buffer = new Byte[10000];
int length = 10000;
int offset = 0;
while (length > 0)
{
length = responseStream.Read(buffer, 0, length);
offset += length;
file.Write(buffer, 0, length);
}
}
catch (Exception e)
{}
finally
{
if(file != null)
file.Close();
if(responseStream != null)
responseStream.Close();
retryAttempt = true;
}
return downloadFileName;
}
StreamReader reader = new StreamReader(responseStream);
responseText = reader.ReadToEnd();
reader.Close();
responseStream.Close();
return responseText;
}
/// <summary>
/// Encode PostBody
/// </summary>
/// <remarks>
/// Encode special characters (such as %, space, <, >, \, and &) to percent values.
/// </remarks>
/// <param name="postbody">Text to be encoded</param>
/// <returns>Encoded text.</returns>
public string EncodePostbody(string postbody)
{
postbody = postbody.Replace(@"%", @"%25");
postbody = postbody.Replace(@" ", @"%20");
postbody = postbody.Replace(@"<", @"%3c");
postbody = postbody.Replace(@">", @"%3e");
postbody = postbody.Replace("\"", @"%22"); // double quote
postbody = postbody.Replace(@"&", @"%26");
return postbody;
}
#endregion
}
}
| {
"pile_set_name": "Github"
} |
# frozen_string_literal: true
lib = File.expand_path('lib', __dir__)
$LOAD_PATH.unshift(lib) unless $LOAD_PATH.include?(lib)
require 'interferon/version'
Gem::Specification.new do |gem|
gem.name = 'interferon'
gem.version = Interferon::VERSION.dup
gem.authors = ['Igor Serebryany', 'Jimmy Ngo']
gem.email = ['igor.serebryany@airbnb.com', 'jimmy.ngo@airbnb.com']
gem.description = %(: Store metrics alerts in code!)
gem.summary = %(: Store metrics alerts in code!)
gem.homepage = 'https://www.github.com/airbnb/interferon'
gem.licenses = ['MIT']
gem.files = `git ls-files`.split($INPUT_RECORD_SEPARATOR)
gem.executables = gem.files.grep(%r{^bin/}).map { |f| File.basename(f) }
gem.test_files = gem.files.grep(%r{^(test|spec|features)/})
gem.add_runtime_dependency 'aws-sdk', '~> 1.35', '>= 1.35.1'
gem.add_runtime_dependency 'diffy', '~> 3.1.0', '>= 3.1.0'
gem.add_runtime_dependency 'dogapi', '~> 1.27', '>= 1.27.0'
gem.add_runtime_dependency 'dogstatsd-ruby', '~> 1.4', '>= 1.4.1'
gem.add_runtime_dependency 'nokogiri', '~> 1.8', '>= 1.8.2'
gem.add_runtime_dependency 'parallel', '~> 1.9', '>= 1.9.0'
gem.add_runtime_dependency 'psych', '~> 2.0'
gem.add_runtime_dependency 'tzinfo', '~> 1.2.2', '>= 1.2.2'
gem.add_development_dependency 'pry', '~> 0.10'
gem.add_development_dependency 'rspec', '~> 3.2'
gem.add_development_dependency 'rubocop', '~> 0.56.0'
end
| {
"pile_set_name": "Github"
} |
# 函数的定义
在PHP中,用户函数的定义从function关键字开始。如下所示简单示例:
[php]
function foo($var) {
echo $var;
}
这是一个非常简单的函数,它所实现的功能是定义一个函数,函数有一个参数,函数的内容是在标准输出端输出传递给它的参数变量的值。
函数的一切从function开始。我们从function开始函数定义的探索之旅。
**词法分析**
在 Zend/zend_language_scanner.l中我们找到如下所示的代码:
[c]
<ST_IN_SCRIPTING>"function" {
return T_FUNCTION;
}
它所表示的含义是function将会生成T_FUNCTION标记。在获取这个标记后,我们开始语法分析。
**语法分析**
在 Zend/zend_language_parser.y文件中找到函数的声明过程标记如下:
[c]
function:
T_FUNCTION { $$.u.opline_num = CG(zend_lineno); }
;
is_reference:
/* empty */ { $$.op_type = ZEND_RETURN_VAL; }
| '&' { $$.op_type = ZEND_RETURN_REF; }
;
unticked_function_declaration_statement:
function is_reference T_STRING {
zend_do_begin_function_declaration(&$1, &$3, 0, $2.op_type, NULL TSRMLS_CC); }
'(' parameter_list ')' '{' inner_statement_list '}' {
zend_do_end_function_declaration(&$1 TSRMLS_CC); }
;
>**NOTE**
>关注点在 function is_reference T_STRING,表示function关键字,是否引用,函数名。
T_FUNCTION标记只是用来定位函数的声明,表示这是一个函数,而更多的工作是与这个函数相关的东西,包括参数,返回值等。
**生成中间代码**
语法解析后,我们看到所执行编译函数为zend_do_begin_function_declaration。在 Zend/zend_complie.c文件中找到其实现如下:
[c]
void zend_do_begin_function_declaration(znode *function_token, znode *function_name,
int is_method, int return_reference, znode *fn_flags_znode TSRMLS_DC) /* {{{ */
{
...//省略
function_token->u.op_array = CG(active_op_array);
lcname = zend_str_tolower_dup(name, name_len);
orig_interactive = CG(interactive);
CG(interactive) = 0;
init_op_array(&op_array, ZEND_USER_FUNCTION, INITIAL_OP_ARRAY_SIZE TSRMLS_CC);
CG(interactive) = orig_interactive;
...//省略
if (is_method) {
...//省略 类方法 在后面的类章节介绍
} else {
zend_op *opline = get_next_op(CG(active_op_array) TSRMLS_CC);
opline->opcode = ZEND_DECLARE_FUNCTION;
opline->op1.op_type = IS_CONST;
build_runtime_defined_function_key(&opline->op1.u.constant, lcname,
name_len TSRMLS_CC);
opline->op2.op_type = IS_CONST;
opline->op2.u.constant.type = IS_STRING;
opline->op2.u.constant.value.str.val = lcname;
opline->op2.u.constant.value.str.len = name_len;
Z_SET_REFCOUNT(opline->op2.u.constant, 1);
opline->extended_value = ZEND_DECLARE_FUNCTION;
zend_hash_update(CG(function_table), opline->op1.u.constant.value.str.val,
opline->op1.u.constant.value.str.len, &op_array, sizeof(zend_op_array),
(void **) &CG(active_op_array));
}
}
/* }}} */
生成的中间代码为 **ZEND_DECLARE_FUNCTION** ,根据这个中间代码及操作数对应的op_type。
我们可以找到中间代码的执行函数为 **ZEND_DECLARE_FUNCTION_SPEC_HANDLER**。
>**NOTE**
>在生成中间代码时,可以看到已经统一了函数名全部为小写,表示函数的名称不是区分大小写的。
为验证这个实现,我们看一段代码:
[php]
function T() {
echo 1;
}
function t() {
echo 2;
}
执行代码,可以看到屏幕上输出如下报错信息:
[shell]
Fatal error: Cannot redeclare t() (previously declared in ...)
表示对于PHP来说T和t是同一个函数名。检验函数名是否重复,这个过程是在哪进行的呢?
下面将要介绍的函数声明中间代码的执行过程包含了这个检查过程。
**执行中间代码**
在 Zend/zend_vm_execute.h 文件中找到 ZEND_DECLARE_FUNCTION中间代码对应的执行函数:ZEND_DECLARE_FUNCTION_SPEC_HANDLER。
此函数只调用了函数do_bind_function。其调用代码为:
[c]
do_bind_function(EX(opline), EG(function_table), 0);
在这个函数中将EX(opline)所指向的函数添加到EG(function_table)中,并判断是否已经存在相同名字的函数,如果存在则报错。
EG(function_table)用来存放执行过程中全部的函数信息,相当于函数的注册表。
它的结构是一个HashTable,所以在do_bind_function函数中添加新的函数使用的是HashTable的操作函数**zend_hash_add**
| {
"pile_set_name": "Github"
} |
/***************************************************************************/
/* */
/* gxvfeat.c */
/* */
/* TrueTypeGX/AAT feat table validation (body). */
/* */
/* Copyright 2004-2018 by */
/* suzuki toshiya, Masatake YAMATO, Red Hat K.K., */
/* David Turner, Robert Wilhelm, and Werner Lemberg. */
/* */
/* This file is part of the FreeType project, and may only be used, */
/* modified, and distributed under the terms of the FreeType project */
/* license, LICENSE.TXT. By continuing to use, modify, or distribute */
/* this file you indicate that you have read the license and */
/* understand and accept it fully. */
/* */
/***************************************************************************/
/***************************************************************************/
/* */
/* gxvalid is derived from both gxlayout module and otvalid module. */
/* Development of gxlayout is supported by the Information-technology */
/* Promotion Agency(IPA), Japan. */
/* */
/***************************************************************************/
#include "gxvalid.h"
#include "gxvcommn.h"
#include "gxvfeat.h"
/*************************************************************************/
/* */
/* The macro FT_COMPONENT is used in trace mode. It is an implicit */
/* parameter of the FT_TRACE() and FT_ERROR() macros, used to print/log */
/* messages during execution. */
/* */
#undef FT_COMPONENT
#define FT_COMPONENT trace_gxvfeat
/*************************************************************************/
/*************************************************************************/
/***** *****/
/***** Data and Types *****/
/***** *****/
/*************************************************************************/
/*************************************************************************/
typedef struct GXV_feat_DataRec_
{
FT_UInt reserved_size;
FT_UShort feature;
FT_UShort setting;
} GXV_feat_DataRec, *GXV_feat_Data;
#define GXV_FEAT_DATA( field ) GXV_TABLE_DATA( feat, field )
typedef enum GXV_FeatureFlagsMask_
{
GXV_FEAT_MASK_EXCLUSIVE_SETTINGS = 0x8000U,
GXV_FEAT_MASK_DYNAMIC_DEFAULT = 0x4000,
GXV_FEAT_MASK_UNUSED = 0x3F00,
GXV_FEAT_MASK_DEFAULT_SETTING = 0x00FF
} GXV_FeatureFlagsMask;
/*************************************************************************/
/*************************************************************************/
/***** *****/
/***** UTILITY FUNCTIONS *****/
/***** *****/
/*************************************************************************/
/*************************************************************************/
static void
gxv_feat_registry_validate( FT_UShort feature,
FT_UShort nSettings,
FT_Bool exclusive,
GXV_Validator gxvalid )
{
GXV_NAME_ENTER( "feature in registry" );
GXV_TRACE(( " (feature = %u)\n", feature ));
if ( feature >= gxv_feat_registry_length )
{
GXV_TRACE(( "feature number %d is out of range %d\n",
feature, gxv_feat_registry_length ));
GXV_SET_ERR_IF_PARANOID( FT_INVALID_DATA );
goto Exit;
}
if ( gxv_feat_registry[feature].existence == 0 )
{
GXV_TRACE(( "feature number %d is in defined range but doesn't exist\n",
feature ));
GXV_SET_ERR_IF_PARANOID( FT_INVALID_DATA );
goto Exit;
}
if ( gxv_feat_registry[feature].apple_reserved )
{
/* Don't use here. Apple is reserved. */
GXV_TRACE(( "feature number %d is reserved by Apple\n", feature ));
if ( gxvalid->root->level >= FT_VALIDATE_TIGHT )
FT_INVALID_DATA;
}
if ( nSettings != gxv_feat_registry[feature].nSettings )
{
GXV_TRACE(( "feature %d: nSettings %d != defined nSettings %d\n",
feature, nSettings,
gxv_feat_registry[feature].nSettings ));
if ( gxvalid->root->level >= FT_VALIDATE_TIGHT )
FT_INVALID_DATA;
}
if ( exclusive != gxv_feat_registry[feature].exclusive )
{
GXV_TRACE(( "exclusive flag %d differs from predefined value\n",
exclusive ));
if ( gxvalid->root->level >= FT_VALIDATE_TIGHT )
FT_INVALID_DATA;
}
Exit:
GXV_EXIT;
}
static void
gxv_feat_name_index_validate( FT_Bytes table,
FT_Bytes limit,
GXV_Validator gxvalid )
{
FT_Bytes p = table;
FT_Short nameIndex;
GXV_NAME_ENTER( "nameIndex" );
GXV_LIMIT_CHECK( 2 );
nameIndex = FT_NEXT_SHORT ( p );
GXV_TRACE(( " (nameIndex = %d)\n", nameIndex ));
gxv_sfntName_validate( (FT_UShort)nameIndex,
255,
32768U,
gxvalid );
GXV_EXIT;
}
static void
gxv_feat_setting_validate( FT_Bytes table,
FT_Bytes limit,
FT_Bool exclusive,
GXV_Validator gxvalid )
{
FT_Bytes p = table;
FT_UShort setting;
GXV_NAME_ENTER( "setting" );
GXV_LIMIT_CHECK( 2 );
setting = FT_NEXT_USHORT( p );
/* If we have exclusive setting, the setting should be odd. */
if ( exclusive && ( setting & 1 ) == 0 )
FT_INVALID_DATA;
gxv_feat_name_index_validate( p, limit, gxvalid );
GXV_FEAT_DATA( setting ) = setting;
GXV_EXIT;
}
static void
gxv_feat_name_validate( FT_Bytes table,
FT_Bytes limit,
GXV_Validator gxvalid )
{
FT_Bytes p = table;
FT_UInt reserved_size = GXV_FEAT_DATA( reserved_size );
FT_UShort feature;
FT_UShort nSettings;
FT_ULong settingTable;
FT_UShort featureFlags;
FT_Bool exclusive;
FT_Int last_setting;
FT_UInt i;
GXV_NAME_ENTER( "name" );
/* feature + nSettings + settingTable + featureFlags */
GXV_LIMIT_CHECK( 2 + 2 + 4 + 2 );
feature = FT_NEXT_USHORT( p );
GXV_FEAT_DATA( feature ) = feature;
nSettings = FT_NEXT_USHORT( p );
settingTable = FT_NEXT_ULONG ( p );
featureFlags = FT_NEXT_USHORT( p );
if ( settingTable < reserved_size )
FT_INVALID_OFFSET;
if ( ( featureFlags & GXV_FEAT_MASK_UNUSED ) == 0 )
GXV_SET_ERR_IF_PARANOID( FT_INVALID_DATA );
exclusive = FT_BOOL( featureFlags & GXV_FEAT_MASK_EXCLUSIVE_SETTINGS );
if ( exclusive )
{
FT_Byte dynamic_default;
if ( featureFlags & GXV_FEAT_MASK_DYNAMIC_DEFAULT )
dynamic_default = (FT_Byte)( featureFlags &
GXV_FEAT_MASK_DEFAULT_SETTING );
else
dynamic_default = 0;
/* If exclusive, check whether default setting is in the range. */
if ( !( dynamic_default < nSettings ) )
FT_INVALID_FORMAT;
}
gxv_feat_registry_validate( feature, nSettings, exclusive, gxvalid );
gxv_feat_name_index_validate( p, limit, gxvalid );
p = gxvalid->root->base + settingTable;
for ( last_setting = -1, i = 0; i < nSettings; i++ )
{
gxv_feat_setting_validate( p, limit, exclusive, gxvalid );
if ( (FT_Int)GXV_FEAT_DATA( setting ) <= last_setting )
GXV_SET_ERR_IF_PARANOID( FT_INVALID_FORMAT );
last_setting = (FT_Int)GXV_FEAT_DATA( setting );
/* setting + nameIndex */
p += ( 2 + 2 );
}
GXV_EXIT;
}
/*************************************************************************/
/*************************************************************************/
/***** *****/
/***** feat TABLE *****/
/***** *****/
/*************************************************************************/
/*************************************************************************/
FT_LOCAL_DEF( void )
gxv_feat_validate( FT_Bytes table,
FT_Face face,
FT_Validator ftvalid )
{
GXV_ValidatorRec gxvalidrec;
GXV_Validator gxvalid = &gxvalidrec;
GXV_feat_DataRec featrec;
GXV_feat_Data feat = &featrec;
FT_Bytes p = table;
FT_Bytes limit = 0;
FT_UInt featureNameCount;
FT_UInt i;
FT_Int last_feature;
gxvalid->root = ftvalid;
gxvalid->table_data = feat;
gxvalid->face = face;
FT_TRACE3(( "validating `feat' table\n" ));
GXV_INIT;
feat->reserved_size = 0;
/* version + featureNameCount + none_0 + none_1 */
GXV_LIMIT_CHECK( 4 + 2 + 2 + 4 );
feat->reserved_size += 4 + 2 + 2 + 4;
if ( FT_NEXT_ULONG( p ) != 0x00010000UL ) /* Version */
FT_INVALID_FORMAT;
featureNameCount = FT_NEXT_USHORT( p );
GXV_TRACE(( " (featureNameCount = %d)\n", featureNameCount ));
if ( !( IS_PARANOID_VALIDATION ) )
p += 6; /* skip (none) and (none) */
else
{
if ( FT_NEXT_USHORT( p ) != 0 )
FT_INVALID_DATA;
if ( FT_NEXT_ULONG( p ) != 0 )
FT_INVALID_DATA;
}
feat->reserved_size += featureNameCount * ( 2 + 2 + 4 + 2 + 2 );
for ( last_feature = -1, i = 0; i < featureNameCount; i++ )
{
gxv_feat_name_validate( p, limit, gxvalid );
if ( (FT_Int)GXV_FEAT_DATA( feature ) <= last_feature )
GXV_SET_ERR_IF_PARANOID( FT_INVALID_FORMAT );
last_feature = GXV_FEAT_DATA( feature );
p += 2 + 2 + 4 + 2 + 2;
}
FT_TRACE4(( "\n" ));
}
/* END */
| {
"pile_set_name": "Github"
} |
/* Modify your Face program from the previous exercise to draw the new output
* shown below. The window size should be changed to 520 x 180 pixels, and the
* faces' top-left corners are at (10, 30), (110, 30), (210, 30), (310, 30),
* and (410, 30).
*/
public class Face2 {
public static void main(String[] args) {
DrawingPanel panel = new DrawingPanel(520, 180);
Graphics g = panel.getGraphics();
for(int i = 0; i < 5; i++)
drawFace(g, 10 + 100 * i, 30);
}
public static void drawFace(Graphics g, int x, int y) {
g.setColor(Color.BLACK);
g.drawOval(x, y, 100, 100); // face outline
g.setColor(Color.BLUE);
g.fillOval(x + 20, y + 30, 20, 20); // eyes
g.fillOval(x + 60, y + 30, 20, 20);
g.setColor(Color.RED); // mouth
g.drawLine(x + 30, y + 70, x + 70, y + 70);
}
}
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="utf-8"?>
<!--
~ Copyright (c) 2016 JustWayward Team
~
~ Licensed under the Apache License, Version 2.0 (the "License");
~ you may not use this file except in compliance with the License.
~ You may obtain a copy of the License at
~
~ http://www.apache.org/licenses/LICENSE-2.0
~
~ Unless required by applicable law or agreed to in writing, software
~ distributed under the License is distributed on an "AS IS" BASIS,
~ WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
~ See the License for the specific language governing permissions and
~ limitations under the License.
-->
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="10dp"
android:gravity="center_vertical"
android:padding="15dp">
<ImageView
android:id="@+id/ivBookCover"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_alignParentLeft="true"
android:layout_centerVertical="true"
android:layout_marginRight="10dp"
android:scaleType="centerInside"
android:src="@drawable/avatar_default" />
<TextView
android:id="@+id/tvBookTitle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toRightOf="@id/ivBookCover"
android:ellipsize="end"
android:singleLine="true"
android:text="我就是静静"
android:textColor="@color/light_coffee"
android:textSize="13sp" />
<TextView
android:id="@+id/tvTime"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/tvBookTitle"
android:layout_marginTop="5dp"
android:layout_toRightOf="@id/ivBookCover"
android:ellipsize="end"
android:singleLine="true"
android:text="昨天"
android:textColor="@color/common_h3" />
</RelativeLayout>
<TextView
android:id="@+id/tvTitle"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:paddingLeft="15dp"
android:paddingRight="15dp"
android:text="1111"
android:textColor="@color/common_h1"
android:textSize="16dp" />
<com.justwayward.reader.view.BookContentTextView
android:id="@+id/tvContent"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:lineSpacingExtra="1dp"
android:paddingLeft="15dp"
android:paddingRight="15dp"
android:text=""
android:textColor="@color/common_h2"
android:textSize="16dp" />
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="15dp">
<TextView
android:id="@+id/tvLike"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/shape_common_btn_selector"
android:gravity="center"
android:paddingBottom="5dp"
android:paddingLeft="10dp"
android:paddingRight="10dp"
android:paddingTop="5dp"
android:text="同感"
android:textColor="@color/colorPrimary"
android:textSize="12dp" />
<ImageView
android:id="@+id/ivMore"
android:layout_width="30dp"
android:layout_height="30dp"
android:layout_alignParentRight="true"
android:src="@drawable/post_detail_more" />
<ImageView
android:id="@+id/ivShare"
android:layout_width="30dp"
android:layout_height="30dp"
android:layout_marginRight="10dp"
android:layout_toLeftOf="@+id/ivMore"
android:src="@drawable/post_detail_share" />
</RelativeLayout>
<TextView
android:id="@+id/tvBestComments"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/common_gray_bg"
android:padding="15dp"
android:text="@string/comment_best_comment"
android:textColor="@color/common_h3"
android:visibility="gone"
tools:visibility="visible" />
<android.support.v7.widget.RecyclerView
android:id="@+id/rvBestComments"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:visibility="gone"
tools:visibility="visible" />
<TextView
android:id="@+id/tvCommentCount"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/common_gray_bg"
android:padding="15dp"
android:text="@string/comment_comment_count"
android:textColor="@color/common_h3" />
</LinearLayout>
| {
"pile_set_name": "Github"
} |
// Copyright 2014 The Go Authors. All rights reserved.
// Use of this source code is governed by a BSD-style
// license that can be found in the LICENSE file.
package icmp
import "syscall"
func init() {
freebsdVersion, _ = syscall.SysctlUint32("kern.osreldate")
}
| {
"pile_set_name": "Github"
} |
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "",
"Effect": "Allow",
"Principal": {
"Federated": "cognito-identity.amazonaws.com"
},
"Action": "sts:AssumeRoleWithWebIdentity",
"Condition": {
"StringEquals": {
"cognito-identity.amazonaws.com:aud": "<IDENTITY_POOL_ID>"
},
"ForAnyValue:StringLike": {
"cognito-identity.amazonaws.com:amr": "unauthenticated"
}
}
}
]
}
| {
"pile_set_name": "Github"
} |
using System.Collections.Generic;
using PlatoCore.Abstractions.Routing;
namespace Plato.Tenants.SignUp
{
public class HomeRoutes : IHomeRouteProvider
{
public IEnumerable<HomeRoute> GetRoutes()
{
return new[]
{
new HomeRoute("Plato.Tenants.SignUp", "Home", "Index"),
};
}
}
}
| {
"pile_set_name": "Github"
} |
<!-- Creator : groff version 1.18.1 -->
<html>
<head>
<meta name="generator" content="groff -Thtml, see www.gnu.org">
<meta name="Content-Style" content="text/css">
<title>AUTOPOINT</title>
</head>
<body>
<h1 align=center>AUTOPOINT</h1>
<a href="#NAME">NAME</a><br>
<a href="#SYNOPSIS">SYNOPSIS</a><br>
<a href="#DESCRIPTION">DESCRIPTION</a><br>
<a href="#OPTIONS">OPTIONS</a><br>
<a href="#AUTHOR">AUTHOR</a><br>
<a href="#REPORTING BUGS">REPORTING BUGS</a><br>
<a href="#SEE ALSO">SEE ALSO</a><br>
<hr>
<a name="NAME"></a>
<h2>NAME</h2>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="10%"></td>
<td width="89%">
<p>autopoint − copies standard gettext
infrastructure</p>
</td>
</table>
<a name="SYNOPSIS"></a>
<h2>SYNOPSIS</h2>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="10%"></td>
<td width="89%">
<p><b>autopoint</b> [<i>OPTION</i>]...</p>
</td>
</table>
<a name="DESCRIPTION"></a>
<h2>DESCRIPTION</h2>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="10%"></td>
<td width="89%">
<p>Copies standard gettext infrastructure files into a
source package.</p>
</td>
</table>
<a name="OPTIONS"></a>
<h2>OPTIONS</h2>
<!-- TABS -->
<table width="100%" border=0 rules="none" frame="void"
cols="5" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="11%"></td>
<td width="8%">
<p><b>−−help</b></p>
</td>
<td width="13%"></td>
<td width="35%">
<p>print this help and exit</p>
</td>
<td width="30%">
</td>
</table>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="10%"></td>
<td width="89%">
<p><b>−−version</b></p></td>
</table>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="21%"></td>
<td width="77%">
<p>print version information and exit</p>
</td>
</table>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="10%"></td>
<td width="89%">
<p><b>−f</b>, <b>−−force</b></p></td>
</table>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="21%"></td>
<td width="77%">
<p>force overwriting of files that already exist</p>
</td>
</table>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="10%"></td>
<td width="89%">
<p><b>−n</b>,
<b>−−dry−run</b></p></td>
</table>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="21%"></td>
<td width="77%">
<p>print modifications but don’t perform them</p>
</td>
</table>
<a name="AUTHOR"></a>
<h2>AUTHOR</h2>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="10%"></td>
<td width="89%">
<p>Written by Bruno Haible</p>
</td>
</table>
<a name="REPORTING BUGS"></a>
<h2>REPORTING BUGS</h2>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="10%"></td>
<td width="89%">
<p>Report bugs to <bug-gnu-gettext@gnu.org>.</p>
<!-- INDENTATION -->
<p>Uses a versions archive in git format. Copyright (C)
2002-2010 Free Software Foundation, Inc. License GPLv3+: GNU
GPL version 3 or later
<http://gnu.org/licenses/gpl.html> This is free
software: you are free to change and redistribute it. There
is NO WARRANTY, to the extent permitted by law.</p>
</td>
</table>
<a name="SEE ALSO"></a>
<h2>SEE ALSO</h2>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="10%"></td>
<td width="89%">
<p>The full documentation for <b>autopoint</b> is maintained
as a Texinfo manual. If the <b>info</b> and <b>autopoint</b>
programs are properly installed at your site, the
command</p>
</td>
</table>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="20%"></td>
<td width="79%">
<p><b>info autopoint</b></p>
</td>
</table>
<!-- INDENTATION -->
<table width="100%" border=0 rules="none" frame="void"
cols="2" cellspacing="0" cellpadding="0">
<tr valign="top" align="left">
<td width="10%"></td>
<td width="89%">
<p>should give you access to the complete manual.</p>
</td>
</table>
<hr>
</body>
</html>
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="utf-8"?>
<!--
VS SDK Notes: This resx file contains the resources that will be consumed from your package by Visual Studio.
For example, Visual Studio will attempt to load resource '400' from this resource stream when it needs to
load your package's icon. Because Visual Studio will always look in the VSPackage.resources stream first for
resources it needs, you should put additional resources that Visual Studio will load directly into this resx
file.
Resources that you would like to access directly from your package in a strong-typed fashion should be stored
in Resources.resx or another resx file.
-->
<root>
<!--
Microsoft ResX Schema
Version 2.0
The primary goals of this format is to allow a simple XML format
that is mostly human readable. The generation and parsing of the
various data types are done through the TypeConverter classes
associated with the data types.
Example:
... ado.net/XML headers & schema ...
<resheader name="resmimetype">text/microsoft-resx</resheader>
<resheader name="version">2.0</resheader>
<resheader name="reader">System.Resources.ResXResourceReader, System.Windows.Forms, ...</resheader>
<resheader name="writer">System.Resources.ResXResourceWriter, System.Windows.Forms, ...</resheader>
<data name="Name1"><value>this is my long string</value><comment>this is a comment</comment></data>
<data name="Color1" type="System.Drawing.Color, System.Drawing">Blue</data>
<data name="Bitmap1" mimetype="application/x-microsoft.net.object.binary.base64">
<value>[base64 mime encoded serialized .NET Framework object]</value>
</data>
<data name="Icon1" type="System.Drawing.Icon, System.Drawing" mimetype="application/x-microsoft.net.object.bytearray.base64">
<value>[base64 mime encoded string representing a byte array form of the .NET Framework object]</value>
<comment>This is a comment</comment>
</data>
There are any number of "resheader" rows that contain simple
name/value pairs.
Each data row contains a name, and value. The row also contains a
type or mimetype. Type corresponds to a .NET class that support
text/value conversion through the TypeConverter architecture.
Classes that don't support this are serialized and stored with the
mimetype set.
The mimetype is used for serialized objects, and tells the
ResXResourceReader how to depersist the object. This is currently not
extensible. For a given mimetype the value must be set accordingly:
Note - application/x-microsoft.net.object.binary.base64 is the format
that the ResXResourceWriter will generate, however the reader can
read any of the formats listed below.
mimetype: application/x-microsoft.net.object.binary.base64
value : The object must be serialized with
: System.Runtime.Serialization.Formatters.Binary.BinaryFormatter
: and then encoded with base64 encoding.
mimetype: application/x-microsoft.net.object.soap.base64
value : The object must be serialized with
: System.Runtime.Serialization.Formatters.Soap.SoapFormatter
: and then encoded with base64 encoding.
mimetype: application/x-microsoft.net.object.bytearray.base64
value : The object must be serialized into a byte array
: using a System.ComponentModel.TypeConverter
: and then encoded with base64 encoding.
-->
<xsd:schema id="root" xmlns="" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:msdata="urn:schemas-microsoft-com:xml-msdata">
<xsd:import namespace="http://www.w3.org/XML/1998/namespace" />
<xsd:element name="root" msdata:IsDataSet="true">
<xsd:complexType>
<xsd:choice maxOccurs="unbounded">
<xsd:element name="metadata">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="value" type="xsd:string" minOccurs="0" />
</xsd:sequence>
<xsd:attribute name="name" use="required" type="xsd:string" />
<xsd:attribute name="type" type="xsd:string" />
<xsd:attribute name="mimetype" type="xsd:string" />
<xsd:attribute ref="xml:space" />
</xsd:complexType>
</xsd:element>
<xsd:element name="assembly">
<xsd:complexType>
<xsd:attribute name="alias" type="xsd:string" />
<xsd:attribute name="name" type="xsd:string" />
</xsd:complexType>
</xsd:element>
<xsd:element name="data">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="value" type="xsd:string" minOccurs="0" msdata:Ordinal="1" />
<xsd:element name="comment" type="xsd:string" minOccurs="0" msdata:Ordinal="2" />
</xsd:sequence>
<xsd:attribute name="name" type="xsd:string" use="required" msdata:Ordinal="1" />
<xsd:attribute name="type" type="xsd:string" msdata:Ordinal="3" />
<xsd:attribute name="mimetype" type="xsd:string" msdata:Ordinal="4" />
<xsd:attribute ref="xml:space" />
</xsd:complexType>
</xsd:element>
<xsd:element name="resheader">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="value" type="xsd:string" minOccurs="0" msdata:Ordinal="1" />
</xsd:sequence>
<xsd:attribute name="name" type="xsd:string" use="required" />
</xsd:complexType>
</xsd:element>
</xsd:choice>
</xsd:complexType>
</xsd:element>
</xsd:schema>
<resheader name="resmimetype">
<value>text/microsoft-resx</value>
</resheader>
<resheader name="version">
<value>2.0</value>
</resheader>
<resheader name="reader">
<value>System.Resources.ResXResourceReader, System.Windows.Forms, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089</value>
</resheader>
<resheader name="writer">
<value>System.Resources.ResXResourceWriter, System.Windows.Forms, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089</value>
</resheader>
<assembly alias="System.Windows.Forms" name="System.Windows.Forms, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" />
<data name="110" xml:space="preserve">
<value>VsTemplateDesigner</value>
</data>
<data name="112" xml:space="preserve">
<value>Information about my package</value>
</data>
<data name="400" type="System.Resources.ResXFileRef, System.Windows.Forms">
<value>Resources\Package.ico;System.Drawing.Icon, System.Drawing, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b03f5f7f11d50a3a</value>
</data>
<data name="100" xml:space="preserve">
<value>[Read Only]</value>
</data>
<data name="101" xml:space="preserve">
<value>File has been changed outside the environment. Reload the new file?</value>
</data>
<data name="102" xml:space="preserve">
<value>VsTemplateDesigner</value>
<comment>Name of the editor to show for the Keybindings</comment>
</data>
<data name="105" xml:space="preserve">
<value>VsTemplateDesigner Files</value>
</data>
<data name="106" xml:space="preserve">
<value>VsTemplateDesigner</value>
</data>
<data name="107" xml:space="preserve">
<value>MyTemplate</value>
</data>
<data name="109" xml:space="preserve">
<value>VsTemplateDesigner File (vstemplate)</value>
</data>
<data name="401" type="System.Resources.ResXFileRef, System.Windows.Forms">
<value>Resources\File.ico;System.Drawing.Icon, System.Drawing, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b03f5f7f11d50a3a</value>
</data>
</root> | {
"pile_set_name": "Github"
} |
require 'spec_helper'
module Bogus
describe OverwritesMethods do
let(:method_stringifier) { MethodStringifier.new }
let(:makes_substitute_methods) { isolate(MakesSubstituteMethods) }
let(:overwriter) { isolate(OverwritesMethods) }
let(:object) { SampleOfOverwriting.new }
context "with regular objects" do
class SampleOfOverwriting
def greet(name)
"Hello #{name}"
end
def wave(part_of_body = "hand", speed = "slowly")
"I'm waving my #{part_of_body} #{speed}"
end
end
before do
overwriter.overwrite(object, :greet)
end
it "does not change the method signature" do
expect(object.method(:greet).arity).to eq(1)
end
it "does not change the method signature" do
expect {
object.greet("John", "Paul")
}.to raise_error(ArgumentError)
end
it "adds interaction recording to the overwritten object" do
object.greet("John")
expect(object).to Bogus.have_received.greet("John")
expect(object).not_to Bogus.have_received.greet("Paul")
end
it "can reset the overwritten methods" do
overwriter.reset(object)
expect(object.greet("John")).to eq("Hello John")
end
it "is imdepotent when overwriting" do
overwriter.overwrite(object, :greet)
overwriter.overwrite(object, :greet)
overwriter.overwrite(object, :greet)
overwriter.reset(object)
expect(object.greet("John")).to eq("Hello John")
end
end
context "with objects that use method missing" do
class UsesMethodMissing
def respond_to?(name)
name == :greet
end
def method_missing(name, *args, &block)
"the original return value"
end
end
let(:object) { UsesMethodMissing.new }
before do
overwriter.overwrite(object, :greet)
end
it "can overwrite the non-existent methods" do
expect(object.methods).to include(:greet)
end
it "can be reset back to the original state" do
overwriter.overwrite(object, :greet)
overwriter.overwrite(object, :greet)
overwriter.reset(object)
expect(object.greet).to eq("the original return value")
end
end
context "with fakes" do
let(:fake) { Samples::FooFake.new }
it "does nothing because fakes methods already work as we need" do
overwriter.overwrite(fake, :foo_bar)
expect(fake).not_to respond_to(:foo_bar)
end
it "does not reset fakes, because there is nothing to reset" do
expect {
overwriter.reset(fake)
}.not_to raise_error
end
end
end
end
| {
"pile_set_name": "Github"
} |
#!/usr/bin/env python
"""
The WiPy firmware update script. Transmits the specified firmware file
over FTP, and then resets the WiPy and optionally verifies that software
was correctly updated.
Usage:
./update-wipy.py --file "path_to_mcuimg.bin" --verify
Or:
python update-wipy.py --file "path_to_mcuimg.bin"
"""
import sys
import argparse
import time
import socket
from ftplib import FTP
from telnetlib import Telnet
def print_exception(e):
print("Exception: {}, on line {}".format(e, sys.exc_info()[-1].tb_lineno))
def ftp_directory_exists(ftpobj, directory_name):
filelist = []
ftpobj.retrlines("LIST", filelist.append)
for f in filelist:
if f.split()[-1] == directory_name:
return True
return False
def transfer_file(args):
with FTP(args.ip, timeout=20) as ftp:
print("FTP connection established")
if "230" in ftp.login(args.user, args.password):
print("Login successful")
if "250" in ftp.cwd("/flash"):
if not ftp_directory_exists(ftp, "sys"):
print("/flash/sys directory does not exist")
if not "550" in ftp.mkd("sys"):
print("/flash/sys directory created")
else:
print("Error: cannot create /flash/sys directory")
return False
if "250" in ftp.cwd("sys"):
print("Entered '/flash/sys' directory")
with open(args.file, "rb") as fwfile:
print("Firmware image found, initiating transfer...")
if "226" in ftp.storbinary("STOR " + "mcuimg.bin", fwfile, 512):
print("File transfer complete")
return True
else:
print("Error: file transfer failed")
else:
print("Error: cannot enter /flash/sys directory")
else:
print("Error: cannot enter /flash directory")
else:
print("Error: ftp login failed")
return False
def reset_board(args):
success = False
try:
tn = Telnet(args.ip, timeout=5)
print("Connected via Telnet, trying to login now")
if b"Login as:" in tn.read_until(b"Login as:", timeout=5):
tn.write(bytes(args.user, "ascii") + b"\r\n")
if b"Password:" in tn.read_until(b"Password:", timeout=5):
# needed because of internal implementation details of the WiPy's telnet server
time.sleep(0.2)
tn.write(bytes(args.password, "ascii") + b"\r\n")
if b'Type "help()" for more information.' in tn.read_until(
b'Type "help()" for more information.', timeout=5
):
print("Telnet login succeeded")
tn.write(b"\r\x03\x03") # ctrl-C twice: interrupt any running program
time.sleep(1)
tn.write(b"\r\x02") # ctrl-B: enter friendly REPL
if b'Type "help()" for more information.' in tn.read_until(
b'Type "help()" for more information.', timeout=5
):
tn.write(b"import machine\r\n")
tn.write(b"machine.reset()\r\n")
time.sleep(2)
print("Reset performed")
success = True
else:
print("Error: cannot enter friendly REPL")
else:
print("Error: telnet login failed")
except Exception as e:
print_exception(e)
finally:
try:
tn.close()
except Exception as e:
pass
return success
def verify_update(args):
success = False
firmware_tag = ""
def find_tag(tag):
if tag in firmware_tag:
print("Verification passed")
return True
else:
print("Error: verification failed, the git tag doesn't match")
return False
retries = 0
while True:
try:
# Specify a longer time out value here because the board has just been
# reset and the wireless connection might not be fully established yet
tn = Telnet(args.ip, timeout=10)
print("Connected via telnet again, lets check the git tag")
break
except socket.timeout:
if retries < 5:
print("Timeout while connecting via telnet, retrying...")
retries += 1
else:
print("Error: Telnet connection timed out!")
return False
try:
firmware_tag = tn.read_until(b"with CC3200")
tag_file_path = args.file.rstrip("mcuimg.bin") + "genhdr/mpversion.h"
if args.tag is not None:
success = find_tag(bytes(args.tag, "ascii"))
else:
with open(tag_file_path) as tag_file:
for line in tag_file:
bline = bytes(line, "ascii")
if b"MICROPY_GIT_HASH" in bline:
bline = (
bline.lstrip(b"#define MICROPY_GIT_HASH ")
.replace(b'"', b"")
.replace(b"\r", b"")
.replace(b"\n", b"")
)
success = find_tag(bline)
break
except Exception as e:
print_exception(e)
finally:
try:
tn.close()
except Exception as e:
pass
return success
def main():
cmd_parser = argparse.ArgumentParser(
description="Update the WiPy firmware with the specified image file"
)
cmd_parser.add_argument("-f", "--file", default=None, help="the path of the firmware file")
cmd_parser.add_argument("-u", "--user", default="micro", help="the username")
cmd_parser.add_argument("-p", "--password", default="python", help="the login password")
cmd_parser.add_argument("--ip", default="192.168.1.1", help="the ip address of the WiPy")
cmd_parser.add_argument(
"--verify", action="store_true", help="verify that the update succeeded"
)
cmd_parser.add_argument("-t", "--tag", default=None, help="git tag of the firmware image")
args = cmd_parser.parse_args()
result = 1
try:
if args.file is None:
raise ValueError("the image file path must be specified")
if transfer_file(args):
if reset_board(args):
if args.verify:
print("Waiting for the WiFi connection to come up again...")
# this time is to allow the system's wireless network card to
# connect to the WiPy again.
time.sleep(5)
if verify_update(args):
result = 0
else:
result = 0
except Exception as e:
print_exception(e)
finally:
sys.exit(result)
if __name__ == "__main__":
main()
| {
"pile_set_name": "Github"
} |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace NoteTaker
{
public class Note
{
public Guid NoteId { get; set; }
public string Text { get; set; }
public DateTime Created { get; set; }
public bool IsUploaded { get; set; }
}
}
| {
"pile_set_name": "Github"
} |
// Copyright 2018 The Chromium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "services/audio/loopback_coordinator.h"
#include "services/audio/group_coordinator-impl.h"
namespace audio {
template class GroupCoordinator<LoopbackGroupMember>;
} // namespace audio
| {
"pile_set_name": "Github"
} |
/*
Copyright 2012 Jun Wako <wakojun@gmail.com>
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 2 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
/*
* scan matrix
*/
#include <stdint.h>
#include <stdbool.h>
#include <string.h>
#include <avr/io.h>
#include <avr/wdt.h>
#include <avr/interrupt.h>
#include <util/delay.h>
#include "print.h"
#include "debug.h"
#include "util.h"
#include "matrix.h"
#include "split_util.h"
#include "quantum.h"
#ifdef USE_MATRIX_I2C
# include "i2c.h"
#else // USE_SERIAL
# include "split_scomm.h"
#endif
#ifndef DEBOUNCE
# define DEBOUNCE 5
#endif
#define ERROR_DISCONNECT_COUNT 5
static uint8_t debouncing = DEBOUNCE;
static const int ROWS_PER_HAND = MATRIX_ROWS/2;
static uint8_t error_count = 0;
uint8_t is_master = 0 ;
static const uint8_t row_pins[MATRIX_ROWS] = MATRIX_ROW_PINS;
static const uint8_t col_pins[MATRIX_COLS] = MATRIX_COL_PINS;
/* matrix state(1:on, 0:off) */
static matrix_row_t matrix[MATRIX_ROWS];
static matrix_row_t matrix_debouncing[MATRIX_ROWS];
static matrix_row_t read_cols(void);
static void init_cols(void);
static void unselect_rows(void);
static void select_row(uint8_t row);
static uint8_t matrix_master_scan(void);
__attribute__ ((weak))
void matrix_init_kb(void) {
matrix_init_user();
}
__attribute__ ((weak))
void matrix_scan_kb(void) {
matrix_scan_user();
}
__attribute__ ((weak))
void matrix_init_user(void) {
}
__attribute__ ((weak))
void matrix_scan_user(void) {
}
inline
uint8_t matrix_rows(void)
{
return MATRIX_ROWS;
}
inline
uint8_t matrix_cols(void)
{
return MATRIX_COLS;
}
void tx_rx_leds_init(void)
{
#ifndef NO_DEBUG_LEDS
setPinOutput(B0);
setPinOutput(D5);
writePinHigh(B0);
writePinHigh(D5);
#endif
}
void tx_led_on(void)
{
#ifndef NO_DEBUG_LEDS
writePinLow(D5);
#endif
}
void tx_led_off(void)
{
#ifndef NO_DEBUG_LEDS
writePinHigh(D5);
#endif
}
void rx_led_on(void)
{
#ifndef NO_DEBUG_LEDS
writePinLow(B0);
#endif
}
void rx_led_off(void)
{
#ifndef NO_DEBUG_LEDS
writePinHigh(B0);
#endif
}
void matrix_init(void)
{
split_keyboard_setup();
// initialize row and col
unselect_rows();
init_cols();
tx_rx_leds_init();
// initialize matrix state: all keys off
for (uint8_t i=0; i < MATRIX_ROWS; i++) {
matrix[i] = 0;
matrix_debouncing[i] = 0;
}
is_master = has_usb();
matrix_init_quantum();
}
uint8_t _matrix_scan(void)
{
// Right hand is stored after the left in the matirx so, we need to offset it
int offset = isLeftHand ? 0 : (ROWS_PER_HAND);
for (uint8_t i = 0; i < ROWS_PER_HAND; i++) {
select_row(i);
_delay_us(30); // without this wait read unstable value.
matrix_row_t cols = read_cols();
if (matrix_debouncing[i+offset] != cols) {
matrix_debouncing[i+offset] = cols;
debouncing = DEBOUNCE;
}
unselect_rows();
}
if (debouncing) {
if (--debouncing) {
_delay_ms(1);
} else {
for (uint8_t i = 0; i < ROWS_PER_HAND; i++) {
matrix[i+offset] = matrix_debouncing[i+offset];
}
}
}
return 1;
}
#ifdef USE_MATRIX_I2C
// Get rows from other half over i2c
int i2c_transaction(void) {
int slaveOffset = (isLeftHand) ? (ROWS_PER_HAND) : 0;
int err = i2c_master_start(SLAVE_I2C_ADDRESS + I2C_WRITE);
if (err) goto i2c_error;
// start of matrix stored at 0x00
err = i2c_master_write(0x00);
if (err) goto i2c_error;
// Start read
err = i2c_master_start(SLAVE_I2C_ADDRESS + I2C_READ);
if (err) goto i2c_error;
if (!err) {
int i;
for (i = 0; i < ROWS_PER_HAND-1; ++i) {
matrix[slaveOffset+i] = i2c_master_read(I2C_ACK);
}
matrix[slaveOffset+i] = i2c_master_read(I2C_NACK);
i2c_master_stop();
} else {
i2c_error: // the cable is disconnceted, or something else went wrong
i2c_reset_state();
return err;
}
return 0;
}
#else // USE_SERIAL
int serial_transaction(int master_changed) {
int slaveOffset = (isLeftHand) ? (ROWS_PER_HAND) : 0;
#ifdef SERIAL_USE_MULTI_TRANSACTION
int ret=serial_update_buffers(master_changed);
#else
int ret=serial_update_buffers();
#endif
if (ret ) {
if(ret==2) rx_led_on();
return 1;
}
rx_led_off();
memcpy(&matrix[slaveOffset],
(void *)serial_slave_buffer, SERIAL_SLAVE_BUFFER_LENGTH);
return 0;
}
#endif
uint8_t matrix_scan(void)
{
if (is_master) {
matrix_master_scan();
}else{
matrix_slave_scan();
int offset = (isLeftHand) ? ROWS_PER_HAND : 0;
memcpy(&matrix[offset],
(void *)serial_master_buffer, SERIAL_MASTER_BUFFER_LENGTH);
matrix_scan_quantum();
}
return 1;
}
uint8_t matrix_master_scan(void) {
int ret = _matrix_scan();
int mchanged = 1;
int offset = (isLeftHand) ? 0 : ROWS_PER_HAND;
#ifdef USE_MATRIX_I2C
// for (int i = 0; i < ROWS_PER_HAND; ++i) {
/* i2c_slave_buffer[i] = matrix[offset+i]; */
// i2c_slave_buffer[i] = matrix[offset+i];
// }
#else // USE_SERIAL
#ifdef SERIAL_USE_MULTI_TRANSACTION
mchanged = memcmp((void *)serial_master_buffer,
&matrix[offset], SERIAL_MASTER_BUFFER_LENGTH);
#endif
memcpy((void *)serial_master_buffer,
&matrix[offset], SERIAL_MASTER_BUFFER_LENGTH);
#endif
#ifdef USE_MATRIX_I2C
if( i2c_transaction() ) {
#else // USE_SERIAL
if( serial_transaction(mchanged) ) {
#endif
// turn on the indicator led when halves are disconnected
tx_led_on();
error_count++;
if (error_count > ERROR_DISCONNECT_COUNT) {
// reset other half if disconnected
int slaveOffset = (isLeftHand) ? (ROWS_PER_HAND) : 0;
for (int i = 0; i < ROWS_PER_HAND; ++i) {
matrix[slaveOffset+i] = 0;
}
}
} else {
// turn off the indicator led on no error
tx_led_off();
error_count = 0;
}
matrix_scan_quantum();
return ret;
}
void matrix_slave_scan(void) {
_matrix_scan();
int offset = (isLeftHand) ? 0 : ROWS_PER_HAND;
#ifdef USE_MATRIX_I2C
for (int i = 0; i < ROWS_PER_HAND; ++i) {
/* i2c_slave_buffer[i] = matrix[offset+i]; */
i2c_slave_buffer[i] = matrix[offset+i];
}
#else // USE_SERIAL
#ifdef SERIAL_USE_MULTI_TRANSACTION
int change = 0;
#endif
for (int i = 0; i < ROWS_PER_HAND; ++i) {
#ifdef SERIAL_USE_MULTI_TRANSACTION
if( serial_slave_buffer[i] != matrix[offset+i] )
change = 1;
#endif
serial_slave_buffer[i] = matrix[offset+i];
}
#ifdef SERIAL_USE_MULTI_TRANSACTION
slave_buffer_change_count += change;
#endif
#endif
}
bool matrix_is_modified(void)
{
if (debouncing) return false;
return true;
}
inline
bool matrix_is_on(uint8_t row, uint8_t col)
{
return (matrix[row] & ((matrix_row_t)1<<col));
}
inline
matrix_row_t matrix_get_row(uint8_t row)
{
return matrix[row];
}
void matrix_print(void)
{
print("\nr/c 0123456789ABCDEF\n");
for (uint8_t row = 0; row < MATRIX_ROWS; row++) {
phex(row); print(": ");
pbin_reverse16(matrix_get_row(row));
print("\n");
}
}
uint8_t matrix_key_count(void)
{
uint8_t count = 0;
for (uint8_t i = 0; i < MATRIX_ROWS; i++) {
count += bitpop16(matrix[i]);
}
return count;
}
static void init_cols(void)
{
for(int x = 0; x < MATRIX_COLS; x++) {
_SFR_IO8((col_pins[x] >> 4) + 1) &= ~_BV(col_pins[x] & 0xF);
_SFR_IO8((col_pins[x] >> 4) + 2) |= _BV(col_pins[x] & 0xF);
}
}
static matrix_row_t read_cols(void)
{
matrix_row_t result = 0;
for(int x = 0; x < MATRIX_COLS; x++) {
result |= (_SFR_IO8(col_pins[x] >> 4) & _BV(col_pins[x] & 0xF)) ? 0 : (1 << x);
}
return result;
}
static void unselect_rows(void)
{
for(int x = 0; x < ROWS_PER_HAND; x++) {
_SFR_IO8((row_pins[x] >> 4) + 1) &= ~_BV(row_pins[x] & 0xF);
_SFR_IO8((row_pins[x] >> 4) + 2) |= _BV(row_pins[x] & 0xF);
}
}
static void select_row(uint8_t row)
{
_SFR_IO8((row_pins[row] >> 4) + 1) |= _BV(row_pins[row] & 0xF);
_SFR_IO8((row_pins[row] >> 4) + 2) &= ~_BV(row_pins[row] & 0xF);
}
| {
"pile_set_name": "Github"
} |
"use strict";
// This icon file is generated automatically.
// tslint:disable
Object.defineProperty(exports, "__esModule", { value: true });
var CaretLeftFilled = { "name": "caret-left", "theme": "filled", "icon": { "tag": "svg", "attrs": { "viewBox": "0 0 1024 1024", "focusable": "false" }, "children": [{ "tag": "path", "attrs": { "d": "M689 165.1L308.2 493.5c-10.9 9.4-10.9 27.5 0 37L689 858.9c14.2 12.2 35 1.2 35-18.5V183.6c0-19.7-20.8-30.7-35-18.5z" } }] } };
exports.default = CaretLeftFilled;
| {
"pile_set_name": "Github"
} |
// Copyright (c)2008-2011, Preferred Infrastructure Inc.
//
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions are met:
//
// * Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// * Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
//
// * Neither the name of Preferred Infrastructure nor the names of other
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
// A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
// OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
// SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
// LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
// DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
// THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
// OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
#ifndef INCLUDE_GUARD_PFI_NETWORK_CGI_SERVER_H_
#define INCLUDE_GUARD_PFI_NETWORK_CGI_SERVER_H_
#include "base.h"
#include "../../lang/shared_ptr.h"
#include "../../concurrent/thread.h"
namespace pfi{
namespace network{
namespace cgi{
class run_server{
public:
typedef pfi::lang::shared_ptr<server_socket> socket_type;
run_server(const cgi &cc, uint16_t port=8080, int thread_num=1, double time_out=10);
run_server(const cgi &cc, socket_type ssock, int thread_num=1);
run_server(const cgi &cc, int argc, char *argv[]);
~run_server();
void run(bool sync=true);
void join();
private:
void process(socket_type ssock,
pfi::lang::shared_ptr<cgi> cc);
void listen(uint16_t port, double time_out);
socket_type ssock;
std::vector<pfi::lang::shared_ptr<pfi::concurrent::thread> > ths;
int thread_num;
const cgi &c;
};
class run_server_or_cgi{
public:
run_server_or_cgi(const cgi &cc, int argc, char *argv[]);
void run();
private:
int argc;
char **argv;
cgi &c;
};
} // cgi
} // network
} // pfi
#endif // #ifndef INCLUDE_GUARD_PFI_NETWORK_CGI_SERVER_H_
| {
"pile_set_name": "Github"
} |
<?php
/*
* This file is part of the Symfony package.
*
* (c) Fabien Potencier <fabien@symfony.com>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Symfony\Component\HttpKernel\Tests\Fixtures;
use Symfony\Component\HttpKernel\Bundle\Bundle;
class FooBarBundle extends Bundle
{
// We need a full namespaced bundle instance to test isClassInActiveBundle
}
| {
"pile_set_name": "Github"
} |
{
"CVE_data_meta": {
"ASSIGNER": "cve@mitre.org",
"ID": "CVE-2018-20698",
"STATE": "PUBLIC"
},
"affects": {
"vendor": {
"vendor_data": [
{
"product": {
"product_data": [
{
"product_name": "n/a",
"version": {
"version_data": [
{
"version_value": "n/a"
}
]
}
}
]
},
"vendor_name": "n/a"
}
]
}
},
"data_format": "MITRE",
"data_type": "CVE",
"data_version": "4.0",
"description": {
"description_data": [
{
"lang": "eng",
"value": "The floragunn Search Guard plugin before 6.x-16 for Kibana allows URL injection for login redirects on the login page when basePath is set."
}
]
},
"problemtype": {
"problemtype_data": [
{
"description": [
{
"lang": "eng",
"value": "n/a"
}
]
}
]
},
"references": {
"reference_data": [
{
"refsource": "CONFIRM",
"name": "https://docs.search-guard.com/latest/changelog-kibana-6.x-16",
"url": "https://docs.search-guard.com/latest/changelog-kibana-6.x-16"
},
{
"refsource": "CONFIRM",
"name": "https://github.com/floragunncom/search-guard-kibana-plugin/pull/140",
"url": "https://github.com/floragunncom/search-guard-kibana-plugin/pull/140"
}
]
}
} | {
"pile_set_name": "Github"
} |
From a10349f1710a11239c58da3a7e5b353c6b2070c2 Mon Sep 17 00:00:00 2001
From: Chaotian Jing <chaotian.jing@mediatek.com>
Date: Mon, 16 Oct 2017 09:46:32 +0800
Subject: [PATCH 153/224] mmc: mediatek: add pad_tune0 support
from mt2701, the register of PAD_TUNE has been phased out,
while there is a new register of PAD_TUNE0
Signed-off-by: Chaotian Jing <chaotian.jing@mediatek.com>
Tested-by: Sean Wang <sean.wang@mediatek.com>
Signed-off-by: Ulf Hansson <ulf.hansson@linaro.org>
---
drivers/mmc/host/mtk-sd.c | 51 ++++++++++++++++++++++++++++++-----------------
1 file changed, 33 insertions(+), 18 deletions(-)
--- a/drivers/mmc/host/mtk-sd.c
+++ b/drivers/mmc/host/mtk-sd.c
@@ -75,6 +75,7 @@
#define MSDC_PATCH_BIT 0xb0
#define MSDC_PATCH_BIT1 0xb4
#define MSDC_PAD_TUNE 0xec
+#define MSDC_PAD_TUNE0 0xf0
#define PAD_DS_TUNE 0x188
#define PAD_CMD_TUNE 0x18c
#define EMMC50_CFG0 0x208
@@ -303,6 +304,7 @@ struct msdc_save_para {
struct mtk_mmc_compatible {
u8 clk_div_bits;
bool hs400_tune; /* only used for MT8173 */
+ u32 pad_tune_reg;
};
struct msdc_tune_para {
@@ -364,21 +366,25 @@ struct msdc_host {
static const struct mtk_mmc_compatible mt8135_compat = {
.clk_div_bits = 8,
.hs400_tune = false,
+ .pad_tune_reg = MSDC_PAD_TUNE,
};
static const struct mtk_mmc_compatible mt8173_compat = {
.clk_div_bits = 8,
.hs400_tune = true,
+ .pad_tune_reg = MSDC_PAD_TUNE,
};
static const struct mtk_mmc_compatible mt2701_compat = {
.clk_div_bits = 12,
.hs400_tune = false,
+ .pad_tune_reg = MSDC_PAD_TUNE0,
};
static const struct mtk_mmc_compatible mt2712_compat = {
.clk_div_bits = 12,
.hs400_tune = false,
+ .pad_tune_reg = MSDC_PAD_TUNE0,
};
static const struct of_device_id msdc_of_ids[] = {
@@ -583,6 +589,7 @@ static void msdc_set_mclk(struct msdc_ho
u32 flags;
u32 div;
u32 sclk;
+ u32 tune_reg = host->dev_comp->pad_tune_reg;
if (!hz) {
dev_dbg(host->dev, "set mclk to 0\n");
@@ -665,10 +672,10 @@ static void msdc_set_mclk(struct msdc_ho
*/
if (host->sclk <= 52000000) {
writel(host->def_tune_para.iocon, host->base + MSDC_IOCON);
- writel(host->def_tune_para.pad_tune, host->base + MSDC_PAD_TUNE);
+ writel(host->def_tune_para.pad_tune, host->base + tune_reg);
} else {
writel(host->saved_tune_para.iocon, host->base + MSDC_IOCON);
- writel(host->saved_tune_para.pad_tune, host->base + MSDC_PAD_TUNE);
+ writel(host->saved_tune_para.pad_tune, host->base + tune_reg);
writel(host->saved_tune_para.pad_cmd_tune,
host->base + PAD_CMD_TUNE);
}
@@ -1226,6 +1233,7 @@ static irqreturn_t msdc_irq(int irq, voi
static void msdc_init_hw(struct msdc_host *host)
{
u32 val;
+ u32 tune_reg = host->dev_comp->pad_tune_reg;
/* Configure to MMC/SD mode, clock free running */
sdr_set_bits(host->base + MSDC_CFG, MSDC_CFG_MODE | MSDC_CFG_CKPDN);
@@ -1241,7 +1249,7 @@ static void msdc_init_hw(struct msdc_hos
val = readl(host->base + MSDC_INT);
writel(val, host->base + MSDC_INT);
- writel(0, host->base + MSDC_PAD_TUNE);
+ writel(0, host->base + tune_reg);
writel(0, host->base + MSDC_IOCON);
sdr_set_field(host->base + MSDC_IOCON, MSDC_IOCON_DDLSEL, 0);
writel(0x403c0046, host->base + MSDC_PATCH_BIT);
@@ -1261,7 +1269,7 @@ static void msdc_init_hw(struct msdc_hos
sdr_set_field(host->base + SDC_CFG, SDC_CFG_DTOC, 3);
host->def_tune_para.iocon = readl(host->base + MSDC_IOCON);
- host->def_tune_para.pad_tune = readl(host->base + MSDC_PAD_TUNE);
+ host->def_tune_para.pad_tune = readl(host->base + tune_reg);
dev_dbg(host->dev, "init hardware done!");
}
@@ -1404,18 +1412,19 @@ static int msdc_tune_response(struct mmc
struct msdc_delay_phase internal_delay_phase;
u8 final_delay, final_maxlen;
u32 internal_delay = 0;
+ u32 tune_reg = host->dev_comp->pad_tune_reg;
int cmd_err;
int i, j;
if (mmc->ios.timing == MMC_TIMING_MMC_HS200 ||
mmc->ios.timing == MMC_TIMING_UHS_SDR104)
- sdr_set_field(host->base + MSDC_PAD_TUNE,
+ sdr_set_field(host->base + tune_reg,
MSDC_PAD_TUNE_CMDRRDLY,
host->hs200_cmd_int_delay);
sdr_clr_bits(host->base + MSDC_IOCON, MSDC_IOCON_RSPL);
for (i = 0 ; i < PAD_DELAY_MAX; i++) {
- sdr_set_field(host->base + MSDC_PAD_TUNE,
+ sdr_set_field(host->base + tune_reg,
MSDC_PAD_TUNE_CMDRDLY, i);
/*
* Using the same parameters, it may sometimes pass the test,
@@ -1439,7 +1448,7 @@ static int msdc_tune_response(struct mmc
sdr_set_bits(host->base + MSDC_IOCON, MSDC_IOCON_RSPL);
for (i = 0; i < PAD_DELAY_MAX; i++) {
- sdr_set_field(host->base + MSDC_PAD_TUNE,
+ sdr_set_field(host->base + tune_reg,
MSDC_PAD_TUNE_CMDRDLY, i);
/*
* Using the same parameters, it may sometimes pass the test,
@@ -1464,12 +1473,12 @@ skip_fall:
final_maxlen = final_fall_delay.maxlen;
if (final_maxlen == final_rise_delay.maxlen) {
sdr_clr_bits(host->base + MSDC_IOCON, MSDC_IOCON_RSPL);
- sdr_set_field(host->base + MSDC_PAD_TUNE, MSDC_PAD_TUNE_CMDRDLY,
+ sdr_set_field(host->base + tune_reg, MSDC_PAD_TUNE_CMDRDLY,
final_rise_delay.final_phase);
final_delay = final_rise_delay.final_phase;
} else {
sdr_set_bits(host->base + MSDC_IOCON, MSDC_IOCON_RSPL);
- sdr_set_field(host->base + MSDC_PAD_TUNE, MSDC_PAD_TUNE_CMDRDLY,
+ sdr_set_field(host->base + tune_reg, MSDC_PAD_TUNE_CMDRDLY,
final_fall_delay.final_phase);
final_delay = final_fall_delay.final_phase;
}
@@ -1477,7 +1486,7 @@ skip_fall:
goto skip_internal;
for (i = 0; i < PAD_DELAY_MAX; i++) {
- sdr_set_field(host->base + MSDC_PAD_TUNE,
+ sdr_set_field(host->base + tune_reg,
MSDC_PAD_TUNE_CMDRRDLY, i);
mmc_send_tuning(mmc, opcode, &cmd_err);
if (!cmd_err)
@@ -1485,7 +1494,7 @@ skip_fall:
}
dev_dbg(host->dev, "Final internal delay: 0x%x\n", internal_delay);
internal_delay_phase = get_best_delay(host, internal_delay);
- sdr_set_field(host->base + MSDC_PAD_TUNE, MSDC_PAD_TUNE_CMDRRDLY,
+ sdr_set_field(host->base + tune_reg, MSDC_PAD_TUNE_CMDRRDLY,
internal_delay_phase.final_phase);
skip_internal:
dev_dbg(host->dev, "Final cmd pad delay: %x\n", final_delay);
@@ -1548,12 +1557,13 @@ static int msdc_tune_data(struct mmc_hos
u32 rise_delay = 0, fall_delay = 0;
struct msdc_delay_phase final_rise_delay, final_fall_delay = { 0,};
u8 final_delay, final_maxlen;
+ u32 tune_reg = host->dev_comp->pad_tune_reg;
int i, ret;
sdr_clr_bits(host->base + MSDC_IOCON, MSDC_IOCON_DSPL);
sdr_clr_bits(host->base + MSDC_IOCON, MSDC_IOCON_W_DSPL);
for (i = 0 ; i < PAD_DELAY_MAX; i++) {
- sdr_set_field(host->base + MSDC_PAD_TUNE,
+ sdr_set_field(host->base + tune_reg,
MSDC_PAD_TUNE_DATRRDLY, i);
ret = mmc_send_tuning(mmc, opcode, NULL);
if (!ret)
@@ -1568,7 +1578,7 @@ static int msdc_tune_data(struct mmc_hos
sdr_set_bits(host->base + MSDC_IOCON, MSDC_IOCON_DSPL);
sdr_set_bits(host->base + MSDC_IOCON, MSDC_IOCON_W_DSPL);
for (i = 0; i < PAD_DELAY_MAX; i++) {
- sdr_set_field(host->base + MSDC_PAD_TUNE,
+ sdr_set_field(host->base + tune_reg,
MSDC_PAD_TUNE_DATRRDLY, i);
ret = mmc_send_tuning(mmc, opcode, NULL);
if (!ret)
@@ -1581,14 +1591,14 @@ skip_fall:
if (final_maxlen == final_rise_delay.maxlen) {
sdr_clr_bits(host->base + MSDC_IOCON, MSDC_IOCON_DSPL);
sdr_clr_bits(host->base + MSDC_IOCON, MSDC_IOCON_W_DSPL);
- sdr_set_field(host->base + MSDC_PAD_TUNE,
+ sdr_set_field(host->base + tune_reg,
MSDC_PAD_TUNE_DATRRDLY,
final_rise_delay.final_phase);
final_delay = final_rise_delay.final_phase;
} else {
sdr_set_bits(host->base + MSDC_IOCON, MSDC_IOCON_DSPL);
sdr_set_bits(host->base + MSDC_IOCON, MSDC_IOCON_W_DSPL);
- sdr_set_field(host->base + MSDC_PAD_TUNE,
+ sdr_set_field(host->base + tune_reg,
MSDC_PAD_TUNE_DATRRDLY,
final_fall_delay.final_phase);
final_delay = final_fall_delay.final_phase;
@@ -1602,6 +1612,7 @@ static int msdc_execute_tuning(struct mm
{
struct msdc_host *host = mmc_priv(mmc);
int ret;
+ u32 tune_reg = host->dev_comp->pad_tune_reg;
if (host->hs400_mode &&
host->dev_comp->hs400_tune)
@@ -1619,7 +1630,7 @@ static int msdc_execute_tuning(struct mm
}
host->saved_tune_para.iocon = readl(host->base + MSDC_IOCON);
- host->saved_tune_para.pad_tune = readl(host->base + MSDC_PAD_TUNE);
+ host->saved_tune_para.pad_tune = readl(host->base + tune_reg);
host->saved_tune_para.pad_cmd_tune = readl(host->base + PAD_CMD_TUNE);
return ret;
}
@@ -1860,10 +1871,12 @@ static int msdc_drv_remove(struct platfo
#ifdef CONFIG_PM
static void msdc_save_reg(struct msdc_host *host)
{
+ u32 tune_reg = host->dev_comp->pad_tune_reg;
+
host->save_para.msdc_cfg = readl(host->base + MSDC_CFG);
host->save_para.iocon = readl(host->base + MSDC_IOCON);
host->save_para.sdc_cfg = readl(host->base + SDC_CFG);
- host->save_para.pad_tune = readl(host->base + MSDC_PAD_TUNE);
+ host->save_para.pad_tune = readl(host->base + tune_reg);
host->save_para.patch_bit0 = readl(host->base + MSDC_PATCH_BIT);
host->save_para.patch_bit1 = readl(host->base + MSDC_PATCH_BIT1);
host->save_para.pad_ds_tune = readl(host->base + PAD_DS_TUNE);
@@ -1873,10 +1886,12 @@ static void msdc_save_reg(struct msdc_ho
static void msdc_restore_reg(struct msdc_host *host)
{
+ u32 tune_reg = host->dev_comp->pad_tune_reg;
+
writel(host->save_para.msdc_cfg, host->base + MSDC_CFG);
writel(host->save_para.iocon, host->base + MSDC_IOCON);
writel(host->save_para.sdc_cfg, host->base + SDC_CFG);
- writel(host->save_para.pad_tune, host->base + MSDC_PAD_TUNE);
+ writel(host->save_para.pad_tune, host->base + tune_reg);
writel(host->save_para.patch_bit0, host->base + MSDC_PATCH_BIT);
writel(host->save_para.patch_bit1, host->base + MSDC_PATCH_BIT1);
writel(host->save_para.pad_ds_tune, host->base + PAD_DS_TUNE);
| {
"pile_set_name": "Github"
} |
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Runtime.InteropServices;
// General Information about an assembly is controlled through the following
// set of attributes. Change these attribute values to modify the information
// associated with an assembly.
[assembly: AssemblyTitle("Budget2.Reports")]
[assembly: AssemblyDescription("")]
[assembly: AssemblyConfiguration("")]
[assembly: AssemblyCompany("Microsoft")]
[assembly: AssemblyProduct("Budget2.Reports")]
[assembly: AssemblyCopyright("Copyright © Microsoft 2010")]
[assembly: AssemblyTrademark("")]
[assembly: AssemblyCulture("")]
// Setting ComVisible to false makes the types in this assembly not visible
// to COM components. If you need to access a type in this assembly from
// COM, set the ComVisible attribute to true on that type.
[assembly: ComVisible(false)]
// The following GUID is for the ID of the typelib if this project is exposed to COM
[assembly: Guid("f227c302-3a75-4f3e-90f1-6426f3fa625c")]
// Version information for an assembly consists of the following four values:
//
// Major Version
// Minor Version
// Build Number
// Revision
//
// You can specify all the values or you can default the Build and Revision Numbers
// by using the '*' as shown below:
// [assembly: AssemblyVersion("1.0.*")]
[assembly: AssemblyVersion("1.0.0.0")]
[assembly: AssemblyFileVersion("1.0.0.0")]
| {
"pile_set_name": "Github"
} |
//
// JPSImagePickerControllerDemoTests.m
// JPSImagePickerControllerDemoTests
//
// Created by JP Simard on 1/31/2014.
// Copyright (c) 2014 JP Simard. All rights reserved.
//
#import <XCTest/XCTest.h>
@interface JPSImagePickerControllerDemoTests : XCTestCase
@end
@implementation JPSImagePickerControllerDemoTests
- (void)setUp
{
[super setUp];
// Put setup code here. This method is called before the invocation of each test method in the class.
}
- (void)tearDown
{
// Put teardown code here. This method is called after the invocation of each test method in the class.
[super tearDown];
}
- (void)testExample
{
XCTFail(@"No implementation for \"%s\"", __PRETTY_FUNCTION__);
}
@end
| {
"pile_set_name": "Github"
} |
/*
Simple DirectMedia Layer
Copyright (C) 1997-2018 Sam Lantinga <slouken@libsdl.org>
This software is provided 'as-is', without any express or implied
warranty. In no event will the authors be held liable for any damages
arising from the use of this software.
Permission is granted to anyone to use this software for any purpose,
including commercial applications, and to alter it and redistribute it
freely, subject to the following restrictions:
1. The origin of this software must not be misrepresented; you must not
claim that you wrote the original software. If you use this software
in a product, an acknowledgment in the product documentation would be
appreciated but is not required.
2. Altered source versions must be plainly marked as such, and must not be
misrepresented as being the original software.
3. This notice may not be removed or altered from any source distribution.
*/
#include "../../SDL_internal.h"
#if SDL_VIDEO_DRIVER_ANDROID
#include "SDL_androidvideo.h"
#include "SDL_androidclipboard.h"
#include "../../core/android/SDL_android.h"
int
Android_SetClipboardText(_THIS, const char *text)
{
return Android_JNI_SetClipboardText(text);
}
char *
Android_GetClipboardText(_THIS)
{
return Android_JNI_GetClipboardText();
}
SDL_bool Android_HasClipboardText(_THIS)
{
return Android_JNI_HasClipboardText();
}
#endif /* SDL_VIDEO_DRIVER_ANDROID */
/* vi: set ts=4 sw=4 expandtab: */
| {
"pile_set_name": "Github"
} |
/*******************************************************************************
* Copyright (c) Microsoft Open Technologies, Inc.
* All Rights Reserved
* Licensed under the Apache License, Version 2.0.
* See License.txt in the project root for license information.
******************************************************************************/
#import "MSOrcEntityFetcher.h"
#import "MSOrcBaseContainer.h"
#import "MSOrcOperations.h"
#import "MSOrcObjectizer.h"
#import "api/MSOrcRequest.h"
#import "api/MSOrcURL.h"
@interface MSOrcEntityFetcher()
@property (copy, nonatomic, readonly) NSString *select;
@property (copy, nonatomic, readonly) NSString *expand;
@end
@implementation MSOrcEntityFetcher
@synthesize operations = _operations;
- (instancetype)initWithUrl:(NSString *)urlComponent
parent:(id<MSOrcExecutable>)parent asClass:(Class)entityClass {
if (self = [super initWithUrl:urlComponent parent:parent asClass:entityClass]) {
_operations = [[MSOrcOperations alloc] initOperationWithUrl:@"" parent:parent];
_expand = nil;
_select = nil;
}
return self;
}
- (void)orcExecuteRequest:(id<MSOrcRequest>)request
callback:(void (^)(id<MSOrcResponse> response, MSOrcError *error))callback {
id<MSOrcURL> url = request.url;
[url appendPathComponent:self.urlComponent];
if (self.select != nil) {
[url addQueryStringParameter:@"$select" value:self.select];
}
if (self.expand != nil) {
[url addQueryStringParameter:@"$expand" value:self.expand];
}
[MSOrcBaseContainer addCustomParametersToOrcURLWithRequest:request
parameters:self.customParameters
headers:self.customHeaders
dependencyResolver:super.resolver];
return [super.parent orcExecuteRequest:request callback:^(id<MSOrcResponse> r, MSOrcError *e) {
callback(r,e);
}];
}
- (void)update:(MSOrcBaseEntity <MSOrcInteroperableWithDictionary> *)entity callback:(void (^)(id updatedEntity, MSOrcError *error))callback {
NSDictionary *updatedValues = [entity toUpdatedValuesDictionary];
NSString *payload = [MSOrcObjectizer deobjectizeToString: updatedValues];
return [self updateRaw:payload callback:^(NSString *response, MSOrcError *e) {
if (e == nil) {
id entity = [MSOrcObjectizer objectizeFromString: response toType: self.entityClass];
callback(entity, e);
}
else {
callback(nil, e);
}
}];
}
- (void)updateRaw:(NSString*)payload
callback:(void (^)(NSString *entityResponse, MSOrcError *error))callback {
id<MSOrcRequest> request = [self.resolver createOrcRequest];
[request setContent:[NSMutableData dataWithData:[payload dataUsingEncoding:NSUTF8StringEncoding]]];
[request setVerb:HTTP_VERB_PATCH];
return [self orcExecuteRequest:request callback:^(id<MSOrcResponse> response, MSOrcError *e) {
if (e == nil) {
callback([[NSString alloc] initWithData:response.data encoding:NSUTF8StringEncoding], e);
}
else {
callback(nil, e);
}
}];
}
-(void)deleteWithCallback:(void (^)(int statusCode, MSOrcError *error))callback {
id<MSOrcRequest> request = [self.resolver createOrcRequest];
[request setVerb:HTTP_VERB_DELETE];
return [self orcExecuteRequest:request callback:^(id<MSOrcResponse> r, MSOrcError *e) {
callback(r.status, e);
}];
}
- (void)readRawWithCallback:(void (^)(NSString *entityString, MSOrcError *error))callback {
id<MSOrcRequest> request = [self.resolver createOrcRequest];
return [self orcExecuteRequest:request callback:^(id<MSOrcResponse> response, MSOrcError *e) {
if (e == nil) {
callback([[NSString alloc] initWithData:response.data encoding:NSUTF8StringEncoding], e);
}
else {
callback(nil, e);
}
}];
}
- (void)readWithCallback:(void (^)(id result, MSOrcError *error))callback {
return [self readRawWithCallback:^(NSString *r, MSOrcError *e) {
if (e == nil) {
id entity = [MSOrcObjectizer objectizeFromString: r toType: self.entityClass];
callback(entity, e);
}
else {
callback(nil, e);
}
}];
}
- (MSOrcEntityFetcher *)select:(NSString *)params {
_select = params;
return self;
}
- (MSOrcEntityFetcher *)expand:(NSString *)value {
_expand = value;
return self;
}
+ (void)setPathForCollectionsWithUrl:(id<MSOrcURL>)url
top:(int)top
skip:(int)skip
select:(NSString *)select
expand:(NSString *)expand
filter:(NSString *)filter
orderby:(NSString *)orderBy
search:(NSString *)search {
if (top > -1) {
[url addQueryStringParameter:@"$top" value:[[NSString alloc] initWithFormat:@"%d", top]];
}
if (skip > -1) {
[url addQueryStringParameter:@"$skip" value:[[NSString alloc] initWithFormat:@"%d", skip]];
}
if (select != nil) {
[url addQueryStringParameter:@"$select" value:select];
}
if (expand != nil) {
[url addQueryStringParameter:@"$expand" value:expand];
}
if (filter!= nil) {
[url addQueryStringParameter:@"$filter" value:filter];
}
if (orderBy != nil) {
[url addQueryStringParameter:@"$orderBy" value:orderBy];
}
if (search != nil) {
[url addQueryStringParameter:@"$search" value:search];
}
}
@end | {
"pile_set_name": "Github"
} |
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<link rel="shortcut icon" href="/favicon.ico" type="image/x-icon" />
<title>$.Object.forEach</title>
<script>
window.$$path = location.protocol + "//" + location.host;
document.write('<script src="' + $$path + '/mass_merge.js"><\/script>')
document.write('<script src="' + $$path + '/doc/scripts/common.js"><\/script>')
</script>
</head>
<body>
<article>
<h3>object.forEach(fn, scope? )</h3>
<p>
<span class="stress">描述:</span>
</p>
<p>对对象的键值使用fn进行迭代。</p>
<dl>
<dt>fn</dt>
<dd>必需。回调函数。</dd>
<dt>score</dt>
<dd>可选。要绑定的作用域。参数依次为value, key。</dd>
</dl>
<fieldset>
<legend>例子</legend>
<pre class="brush:javascript;gutter:false;toolbar:false">
$.require("lang", function() {
var obj = {
first: 'Sunday',
second: 'Monday',
third: 'Tuesday'
};
$.Object.forEach(obj, function(v) {
$.log(v, true);
});
});
</pre>
<button class="doc_btn" type="button">点我,执行代码</button>
</fieldset>
</article>
</body>
</html> | {
"pile_set_name": "Github"
} |
---
title: Namespaces for WIA Drivers
description: Namespaces for WIA Drivers
ms.assetid: 67260a25-6233-4738-a08f-26223cc8e563
ms.date: 04/20/2017
ms.localizationpriority: medium
---
# Namespaces for WIA Drivers
All services run in session zero. However, applications might be running in a different session. Each session has its own *namespace*. Therefore, a named object created in one session will not generally be visible to a component in another session.
The solution to this problem is to ensure that both components use the same namespace. The simplest way to do this is to use the *global namespace*. For example, if a bundled component were to access a device outside of WIA, it might use a mutex object named **MyDeviceLock** to synchronize access with its WIA driver. In order to put the mutex name in the global namespace, it should be called **Global\\MyDeviceLock**. The mutex named **Global\\MyDeviceLock** is visible to both the driver and the application, no matter which sessions they are running in, because they both specify that the name belongs to the global namespace.
See "Kernel Object Name Spaces" in the Microsoft Windows SDK documentation for more information.
| {
"pile_set_name": "Github"
} |
/* -*- Mode: C++; tab-width: 4; indent-tabs-mode: nil; c-basic-offset: 4 -*- */
/*
* This file is part of the LibreOffice project.
*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*
* This file incorporates work covered by the following license notice:
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed
* with this work for additional information regarding copyright
* ownership. The ASF licenses this file to you under the Apache
* License, Version 2.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a copy of
* the License at http://www.apache.org/licenses/LICENSE-2.0 .
*/
#ifndef com_sun_star_chart2_data_XNumericalDataSequence_idl
#define com_sun_star_chart2_data_XNumericalDataSequence_idl
#include <com/sun/star/chart2/data/XDataSequence.idl>
module com
{
module sun
{
module star
{
module chart2
{
module data
{
/** allows access to a one-dimensional sequence of double precision
floating-point numbers.
*/
interface XNumericalDataSequence : ::com::sun::star::uno::XInterface
{
/** retrieves data as `double` values.
*/
sequence< double > getNumericalData();
};
} ; // data
} ; // chart2
} ; // com
} ; // sun
} ; // star
#endif
/* vim:set shiftwidth=4 softtabstop=4 expandtab: */
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="utf-8"?>
<Project DefaultTargets="Build" ToolsVersion="4.0" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<PropertyGroup>
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
<Platform Condition=" '$(Platform)' == '' ">x86</Platform>
<ProjectGuid>{C34D6D75-6F2F-4F5A-968D-3B2D1F0E290B}</ProjectGuid>
<OutputType>Exe</OutputType>
<RootNamespace>Cons</RootNamespace>
<AssemblyName>Cons</AssemblyName>
<TargetFrameworkVersion>v4.5</TargetFrameworkVersion>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|x86' ">
<DebugSymbols>true</DebugSymbols>
<DebugType>full</DebugType>
<Optimize>false</Optimize>
<OutputPath>bin\Debug</OutputPath>
<DefineConstants>DEBUG;</DefineConstants>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
<ExternalConsole>true</ExternalConsole>
<PlatformTarget>x86</PlatformTarget>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|x86' ">
<Optimize>true</Optimize>
<OutputPath>bin\Release</OutputPath>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
<ExternalConsole>true</ExternalConsole>
<PlatformTarget>x86</PlatformTarget>
</PropertyGroup>
<ItemGroup>
<Reference Include="System, Version=1.0.5000.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" />
</ItemGroup>
<ItemGroup>
<Compile Include="Program.cs" />
<Compile Include="Properties\AssemblyInfo.cs" />
</ItemGroup>
<Import Project="$(MSBuildBinPath)\Microsoft.CSharp.targets" />
</Project> | {
"pile_set_name": "Github"
} |
package com.phoenixnap.oss.ramlplugin.raml2code.github;
import org.junit.Test;
import com.phoenixnap.oss.ramlplugin.raml2code.rules.GitHubAbstractRuleTestBase;
import com.phoenixnap.oss.ramlplugin.raml2code.rules.Spring4ControllerDecoratorRule;
/**
* @author aleksandars
* @since 0.10.13
*/
public class Issue215RulesTest extends GitHubAbstractRuleTestBase {
@Test
public void optional_param_as_resource_level() throws Exception {
loadRaml("issue-215-1.raml");
rule = new Spring4ControllerDecoratorRule();
rule.apply(getControllerMetadata(), jCodeModel);
verifyGeneratedCode("Issue215-1Spring4ControllerStub");
}
@Test
public void optional_param_as_resource_part() throws Exception {
loadRaml("issue-215-2.raml");
rule = new Spring4ControllerDecoratorRule();
rule.apply(getControllerMetadata(), jCodeModel);
verifyGeneratedCode("Issue215-2Spring4ControllerStub");
}
@Test
public void two_optional_parms() throws Exception {
loadRaml("issue-215-3.raml");
rule = new Spring4ControllerDecoratorRule();
rule.apply(getControllerMetadata(), jCodeModel);
verifyGeneratedCode("Issue215-3Spring4ControllerStub");
}
}
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="UTF-8"?>
<!--
Syntax highlighting definition for JavaScript
xslthl - XSLT Syntax Highlighting
http://sourceforge.net/projects/xslthl/
Copyright (C) 2005-2008 Michal Molhanec, Jirka Kosek, Michiel Hendriks
This software is provided 'as-is', without any express or implied
warranty. In no event will the authors be held liable for any damages
arising from the use of this software.
Permission is granted to anyone to use this software for any purpose,
including commercial applications, and to alter it and redistribute it
freely, subject to the following restrictions:
1. The origin of this software must not be misrepresented; you must not
claim that you wrote the original software. If you use this software
in a product, an acknowledgment in the product documentation would be
appreciated but is not required.
2. Altered source versions must be plainly marked as such, and must not be
misrepresented as being the original software.
3. This notice may not be removed or altered from any source distribution.
Michal Molhanec <mol1111 at users.sourceforge.net>
Jirka Kosek <kosek at users.sourceforge.net>
Michiel Hendriks <elmuerte at users.sourceforge.net>
-->
<highlighters>
<highlighter type="multiline-comment">
<start>/*</start>
<end>*/</end>
</highlighter>
<highlighter type="oneline-comment">//</highlighter>
<highlighter type="string">
<string>"</string>
<escape>\</escape>
</highlighter>
<highlighter type="string">
<string>'</string>
<escape>\</escape>
</highlighter>
<highlighter type="hexnumber">
<prefix>0x</prefix>
<ignoreCase />
</highlighter>
<highlighter type="number">
<point>.</point>
<exponent>e</exponent>
<ignoreCase />
</highlighter>
<highlighter type="keywords">
<keyword>break</keyword>
<keyword>case</keyword>
<keyword>catch</keyword>
<keyword>continue</keyword>
<keyword>default</keyword>
<keyword>delete</keyword>
<keyword>do</keyword>
<keyword>else</keyword>
<keyword>finally</keyword>
<keyword>for</keyword>
<keyword>function</keyword>
<keyword>if</keyword>
<keyword>in</keyword>
<keyword>instanceof</keyword>
<keyword>new</keyword>
<keyword>return</keyword>
<keyword>switch</keyword>
<keyword>this</keyword>
<keyword>throw</keyword>
<keyword>try</keyword>
<keyword>typeof</keyword>
<keyword>var</keyword>
<keyword>void</keyword>
<keyword>while</keyword>
<keyword>with</keyword>
<!-- future keywords -->
<keyword>abstract</keyword>
<keyword>boolean</keyword>
<keyword>byte</keyword>
<keyword>char</keyword>
<keyword>class</keyword>
<keyword>const</keyword>
<keyword>debugger</keyword>
<keyword>double</keyword>
<keyword>enum</keyword>
<keyword>export</keyword>
<keyword>extends</keyword>
<keyword>final</keyword>
<keyword>float</keyword>
<keyword>goto</keyword>
<keyword>implements</keyword>
<keyword>import</keyword>
<keyword>int</keyword>
<keyword>interface</keyword>
<keyword>long</keyword>
<keyword>native</keyword>
<keyword>package</keyword>
<keyword>private</keyword>
<keyword>protected</keyword>
<keyword>public</keyword>
<keyword>short</keyword>
<keyword>static</keyword>
<keyword>super</keyword>
<keyword>synchronized</keyword>
<keyword>throws</keyword>
<keyword>transient</keyword>
<keyword>volatile</keyword>
</highlighter>
<highlighter type="keywords">
<keyword>prototype</keyword>
<!-- Global Objects -->
<keyword>Array</keyword>
<keyword>Boolean</keyword>
<keyword>Date</keyword>
<keyword>Error</keyword>
<keyword>EvalError</keyword>
<keyword>Function</keyword>
<keyword>Math</keyword>
<keyword>Number</keyword>
<keyword>Object</keyword>
<keyword>RangeError</keyword>
<keyword>ReferenceError</keyword>
<keyword>RegExp</keyword>
<keyword>String</keyword>
<keyword>SyntaxError</keyword>
<keyword>TypeError</keyword>
<keyword>URIError</keyword>
<!-- Global functions -->
<keyword>decodeURI</keyword>
<keyword>decodeURIComponent</keyword>
<keyword>encodeURI</keyword>
<keyword>encodeURIComponent</keyword>
<keyword>eval</keyword>
<keyword>isFinite</keyword>
<keyword>isNaN</keyword>
<keyword>parseFloat</keyword>
<keyword>parseInt</keyword>
<!-- Global properties -->
<keyword>Infinity</keyword>
<keyword>NaN</keyword>
<keyword>undefined</keyword>
</highlighter>
</highlighters> | {
"pile_set_name": "Github"
} |
RamlDocumentNode (Start: 11 , End: 65, On: input.raml, Source: SYObjectNode)
KeyValueNodeImpl (Start: 11 , End: 22, On: input.raml)
SYStringNode: "title" (Start: 11 , End: 16, On: input.raml)
OverlayableObjectNodeImpl (Start: 18 , End: 22, On: input.raml, Source: SYStringNode)
KeyValueNodeImpl (Start: 11 , End: 22, On: input.raml)
StringNodeImpl: "value" (Start: -1 , End: -1)
OverlayableStringNode: "hola" (Start: 18 , End: 22, On: input.raml)
ResourceNode (Start: 23 , End: 47, On: input.raml, Source: KeyValueNodeImpl)
SYStringNode: "/top" (Start: 23 , End: 27, On: input.raml)
SYObjectNode (Start: 0 , End: 47, On: subdir/basic-resource.raml, Source: SYIncludeNode)
MethodNode (Start: 0 , End: 24, On: subdir/basic-resource.raml, Source: KeyValueNodeImpl)
SYStringNode: "get" (Start: 0 , End: 3, On: subdir/basic-resource.raml)
SYObjectNode (Start: 0 , End: 24, On: subdir/sub2/action.raml, Source: SYIncludeNode)
KeyValueNodeImpl (Start: 0 , End: 24, On: subdir/sub2/action.raml)
SYStringNode: "description" (Start: 0 , End: 11, On: subdir/sub2/action.raml)
OverlayableObjectNodeImpl (Start: 13 , End: 24, On: subdir/sub2/action.raml, Source: SYStringNode)
KeyValueNodeImpl (Start: 0 , End: 24, On: subdir/sub2/action.raml)
StringNodeImpl: "value" (Start: -1 , End: -1)
OverlayableStringNode: "description" (Start: 13 , End: 24, On: subdir/sub2/action.raml)
KeyValueNodeImpl (Start: -1 , End: -1)
StringNodeImpl: "displayName" (Start: -1 , End: -1)
ObjectNodeImpl (Start: -1 , End: -1)
KeyValueNodeImpl (Start: -1 , End: -1)
StringNodeImpl: "value" (Start: -1 , End: -1)
OverlayableStringNode: "get" (Start: -1 , End: -1, Source: SYStringNode)
ResourceNode (Start: 31 , End: 47, On: subdir/basic-resource.raml, Source: KeyValueNodeImpl)
SYStringNode: "/{id}" (Start: 31 , End: 36, On: subdir/basic-resource.raml)
SYObjectNode (Start: 42 , End: 47, On: subdir/basic-resource.raml)
MethodNode (Start: 42 , End: 46, On: subdir/basic-resource.raml, Source: KeyValueNodeImpl)
SYStringNode: "put" (Start: 42 , End: 45, On: subdir/basic-resource.raml)
ObjectNodeImpl (Start: 46 , End: 46, On: subdir/basic-resource.raml, Source: SYNullNode)
KeyValueNodeImpl (Start: -1 , End: -1)
StringNodeImpl: "displayName" (Start: -1 , End: -1)
ObjectNodeImpl (Start: -1 , End: -1)
KeyValueNodeImpl (Start: -1 , End: -1)
StringNodeImpl: "value" (Start: -1 , End: -1)
OverlayableStringNode: "put" (Start: -1 , End: -1, Source: SYStringNode)
KeyValueNodeImpl (Start: -1 , End: -1)
StringNodeImpl: "displayName" (Start: -1 , End: -1)
ObjectNodeImpl (Start: -1 , End: -1)
KeyValueNodeImpl (Start: -1 , End: -1)
StringNodeImpl: "value" (Start: -1 , End: -1)
OverlayableStringNode: "/{id}" (Start: -1 , End: -1, Source: SYStringNode)
KeyValueNodeImpl (Start: -1 , End: -1)
StringNodeImpl: "uriParameters" (Start: -1 , End: -1)
ObjectNodeImpl (Start: -1 , End: -1)
PropertyNode (Start: -1 , End: -1)
StringNodeImpl: "id" (Start: -1 , End: -1)
TypeDeclarationNode (Start: -1 , End: -1, Source: NativeTypeExpressionNode)
KeyValueNodeImpl (Start: -1 , End: -1)
StringNodeImpl: "type" (Start: -1 , End: -1)
NativeTypeExpressionNode: "string" (Start: -1 , End: -1, Source: NativeTypeExpressionNode)
KeyValueNodeImpl (Start: -1 , End: -1)
StringNodeImpl: "displayName" (Start: -1 , End: -1)
ObjectNodeImpl (Start: -1 , End: -1)
KeyValueNodeImpl (Start: -1 , End: -1)
StringNodeImpl: "value" (Start: -1 , End: -1)
OverlayableStringNode: "/top" (Start: -1 , End: -1, Source: SYStringNode)
| {
"pile_set_name": "Github"
} |
# MLIR: The case for a simplified polyhedral form
MLIR embraces polyhedral compiler techniques for their many advantages
representing and transforming dense numerical kernels, but it uses a form that
differs significantly from other polyhedral frameworks.
**Disclaimer / Warning**
This document is a very early design proposal (which has since been accepted)
that explored the tradeoffs of using this simplified form vs the traditional
polyhedral schedule list form. At some point, this document could be dusted off
and written as a proper academic paper, but until now, it is better to included
it in this crafty form than not to. Beware that this document uses archaic
syntax and should not be considered a canonical reference to modern MLIR.
## Introduction
This document discusses general goals of the project, introduces context and the
two alternatives, then talks about the tradeoffs of these designs. Written by
Chris Lattner.
## General goals of an IR, and goals of mlfunc's specifically
Our currently planned representation for MLIR consists of two kinds of
functions: an LLVM-like "CFG Function" and an "ML Function": a function
represented in multidimensional loop form. The idea is that a CFG function is
capable of full generality for expressing arbitrary computation, but is awkward
for loop transformations. In contrast, mlfunc's are limited (e.g. to control
flow involving loop nests over affine spaces) but these limitations make it much
easier to transform and analyze, particularly for the set of computations in a
machine learning kernel.
The design of an intermediate representations is an optimization problem, which
makes intentional tradeoffs that aim to make certain kinds of compiler
transformations simple. After all, it is "possible" to do almost any
transformation on any IR: we could theoretically do loop transformations on
assembly language. OTOH, such transformations would take too long to write,
would be fragile due to irrelevant changes, would be difficult to maintain, and
difficult to make target independent. Performing transformations on the "right
level" of IR makes it much easier to do analysis and transformation of code, and
can make them faster by reducing the size of the IR, and eliminating
possibilities that would have otherwise have to be considered.
This is the reason we're interested in adding polyhedral techniques to an IR in
the first place: though our base "CFG function" representation is fully capable
of expressing any computation, it is "too" expressive. The limitations imposed
by polyhedral techniques (e.g. on affine loop bounds and array subscripts)
define a closed algebra that can represent an interesting range of
transformations and their compositions, and because of their simplicity, we can
perform (e.g.) dependence analysis more efficiently and more reliably.
This raises an important question that this document examines: given we are
introducing a redundant and limited way to express code and transformations,
exactly what form is best to perform the analyses and transformations we want?
We explore two different design points that are capable of expressing the same
class of affine loop computations, but which use different representational
forms. These forms trade off verbosity, ease of transformation, and ease of
analysis in interesting ways.
## Context: Traditional Polyhedral Form
We started by discussing a representation that uses the traditional polyhedral
schedule set + domain representation, e.g. consider C-like code like:
```c
void simple_example(...) {
for (int i = 0; i < N; ++i) {
for (int j = 0; j < N; ++j) {
float tmp = X[i,j] // S1
A[i,j] = tmp + 1 // S2
B[i,j] = tmp * 42 // S3
}
}
}
```
The polyhedral representation doesn't care about the actual computation, so we
will abstract them into S1/S2/S3 in the discussion below. Originally, we planned
to represent this with a classical form like (syntax details are not important
and probably slightly incorrect below):
```mlir
mlfunc @simple_example(... %N) {
%tmp = call @S1(%X, %i, %j)
domain: (0 <= %i < %N), (0 <= %j < %N)
schedule: (i, j, 0)
call @S2(%tmp, %A, %i, %j)
domain: (0 <= %i < %N), (0 <= %j < %N)
schedule: (i, j, 1)
call @S3(%tmp, %B, %i, %j)
domain: (0 <= %i < %N), (0 <= %j < %N)
schedule: (i, j, 2)
}
```
In this design, an mlfunc is an unordered bag of instructions whose execution
order is fully controlled by their schedule.
However, we recently agreed that a more explicit schedule tree representation is
a better fit for our needs, because it exposes important structure that will
make analyses and optimizations more efficient, and also makes the scoping of
SSA values more explicit. This leads us to a representation along the lines of:
```mlir
mlfunc @simple_example(... %N) {
d0/d1 = mlspace
for S1(d0), S2(d0), S3(d0) {
for S1(d1), S2(d1), S3(d1) {
%tmp = call @S1(%X, d0, d1) ;; S1
domain: (0 <= d0 < %N), (0 <= d1 < %N)
call @S2(%tmp, %A, d0, d1) ;; S2
domain: (0 <= d0 < %N), (0 <= d1 < %N)
call @S3(%tmp, %B, d0, d1) ;; S3
domain: (0 <= d0 < %N), (0 <= d1 < %N)
}
}
}
```
This change makes the nesting structure of the loops an explicit part of the
representation, and makes lexical ordering within a loop significant
(eliminating the constant 0/1/2 of schedules).
It isn't obvious in the example above, but the representation allows for some
interesting features, including the ability for instructions within a loop nest
to have non-equal domains, like this - the second instruction ignores the outer
10 points inside the loop:
```mlir
mlfunc @reduced_domain_example(... %N) {
d0/d1 = mlspace
for S1(d0), S2(d0) {
for S1(d1), S2(d1) {
%tmp = call @S1(%X, d0, d1) ;; S1
domain: (0 <= d0 < %N), (0 <= d1 < %N)
call @S2(%tmp, %A, d0, d1) ;; S2
domain: (10 <= d0 < %N-10), (10 <= d1 < %N-10)
}
}
}
```
It also allows schedule remapping within the instruction, like this example that
introduces a diagonal skew through a simple change to the schedules of the two
instructions:
```mlir
mlfunc @skewed_domain_example(... %N) {
d0/d1 = mlspace
for S1(d0), S2(d0+d1) {
for S1(d0+d1), S2(d1) {
%tmp = call @S1(%X, d0, d1) ;; S1
domain: (0 <= d0 < %N), (0 <= d1 < %N)
call @S2(%tmp, %A, d0, d1) ;; S2
domain: (0 <= d0 < %N), (0 <= d1 < %N)
}
}
}
```
This form has great power, and the polyhedral code generator (which lowers from
an mlfunc to a cfgfunc representation) handles this power so things that
introduce loop transformations don't have to explicitly manipulate the looping
structure.
## Proposal: Simplified Polyhedral Form
This document proposes and explores the idea of going one step further, moving
all of the domain and schedule information into the "schedule tree". In this
form, we would have a representation where all instructions inside of a given
for-loop are known to have the same domain, which is maintained by the loop. In
the simplified form, we also have an "if" instruction that takes an affine
condition.
Our simple example above would be represented as:
```mlir
mlfunc @simple_example(... %N) {
affine.for %i = 0 ... %N step 1 {
affine.for %j = 0 ... %N step 1 {
// identity noop in this case, but can exist in general.
%0,%1 = affine.apply #57(%i, %j)
%tmp = call @S1(%X, %0, %1)
call @S2(%tmp, %A, %0, %1)
call @S3(%tmp, %B, %0, %1)
}
}
}
```
The example with the reduced domain would be represented with an if instruction:
```mlir
mlfunc @reduced_domain_example(... %N) {
affine.for %i = 0 ... %N step 1 {
affine.for %j = 0 ... %N step 1 {
// identity noop in this case, but can exist in general.
%0,%1 = affinecall #57(%i, %j)
%tmp = call @S1(%X, %0, %1)
if (10 <= %i < %N-10), (10 <= %j < %N-10) {
%2,%3 = affine.apply(%i, %j) // identity noop in this case
call @S2(%tmp, %A, %2, %3)
}
}
}
}
```
These IRs represent exactly the same information, and use a similar information
density. The 'traditional' form introduces an extra level of abstraction
(schedules and domains) that make it easy to transform instructions at the
expense of making it difficult to reason about how those instructions will come
out after code generation. With the simplified form, transformations have to do
parts of code generation inline with their transformation: instead of simply
changing a schedule to **(i+j, j)** to get skewing, you'd have to generate this
code explicitly (potentially implemented by making polyhedral codegen a library
that transformations call into):
```mlir
mlfunc @skewed_domain_example(... %N) {
affine.for %t1 = 0 ... 2*N-2 step 1 {
affine.for %t2 = max(0, t1-N+1) ... min(N, t1) step 1 {
(%i, %j) = (%t1-%t2, %t2)
...
}
}
}
```
## Evaluation
Both of these forms are capable of expressing the same class of computation:
multidimensional loop nests with affine loop bounds and affine memory
references. That said, they pose very different tradeoffs in other ways.
### Commonality: can express same computation
Both of these can express the same sorts of computation, e.g. kernels written in
one form are representable in the other form in all cases.
### Commonality: dependence analysis
These representations both use affine functions for data layout mapping and
access subscripts, and dependence analysis works the same way.
### Commonality: difficulty of determining optimal transformation series
One major challenge in performance of optimization of this sort of code is
choosing the ordering and behavior of various loop transformations that get
applied. There are non-local effects of every decision, and neither
representation helps solve this inherently hard problem.
### Commonality: compactness of IR
In the cases that are most relevant to us (hyper rectangular spaces) these forms
are directly equivalent: a traditional instruction with a limited domain (e.g.
the "reduced_domain_example" above) ends up having one level of ML 'if' inside
its loops. The simplified form pays for this by eliminating schedules and
domains from the IR. Both forms allow code duplication to reduce dynamic
branches in the IR: the traditional approach allows instruction splitting, the
simplified form supports instruction duplication.
It is important to point out that the traditional form wins on compactness in
the extreme cases: e.g. the loop skewing case. These cases will be rare in
practice for our workloads, and are exactly the cases that downstream
transformations want to be explicit about what they are doing.
### Simplicity of code generation
A key final stage of an mlfunc is its conversion to a CFG function, which is
required as part of lowering to the target machine. The simplified form has a
clear advantage here: the IR has a direct correspondence to the structure of the
generated code.
In contrast, the traditional form has significant complexity in the lowering
process to a CFG function, because the verbosity not imbued in the IR needs to
come out during code generation. Code generation from ISL shows that it is
possible to do this, but it is a non-trivial transformation.
### Ease of transformation
An advantage for the traditional form is that it is easier to perform certain
transformations on it: skewing and tiling are just transformations on the
schedule of the instructions in question, it doesn't require changing the loop
structure.
In practice, the simplified form requires moving the complexity of code
generation into the transformations themselves - this is sometimes trivial,
sometimes involved. The author believes that this should be possible by making
the code generation algorithms themselves be library functions that
transformations call into, instead of an opaque block that happens at the end of
the mlfunc processing.
Also, the sorts of transformations performed today by XLA (including tiling,
padding, unrolling, and other rectangular transformations) should be easy enough
to implement on either representation. The only cases that are a challenge are
more advanced cases like skewing, e.g. for DMA data movement generation.
### Ease of analysis: Cost models
The simplified form is much easier for analyses and transformations to build
cost models for (e.g. answering the question of "how much code bloat will be
caused by unrolling a loop at this level?"), because it is easier to predict
what target code will be generated. With the traditional form, these analyses
will have to anticipate what polyhedral codegen will do to a set of instructions
under consideration: something that is non-trivial in the interesting cases in
question (see "Cost of code generation").
### Cost of code generation
State of the art polyhedral code generation is
[expensive and complicated](https://lirias.kuleuven.be/bitstream/123456789/497238/1/toplas-astgen.pdf),
sometimes exponential time complexity. We expect that most machine learning
workloads will be hyper-rectangular, and thus it should be easy to specialize in
important cases. That said, the traditional polyhedral representation makes it
very easy to introduce complicated and expensive schedules, and provides no way
to understand and project a cost model for using them. All downstream clients of
the IR need to be prepared to handle the full generality of IR that may come to
them.
The simplified form defines this away: the concepts in the IR remain simple, and
the code much more directly reflects the cost model for lowering to CFG
functions and machine code. This is expected to be very important in the late
stages of a code generator for an accelerator.
### SSA in ML Functions
We agree already that values defined in an mlfunc can include scalar values and
they are defined based on traditional dominance. In the simplified form, this is
very simple: arguments and induction variables defined in for-loops are live
inside their lexical body, and linear series of instructions have the same "top
down" dominance relation that a basic block does.
In the traditional form though, this is not the case: it seems that a lot of
knowledge about how codegen will emit the code is necessary to determine if SSA
form is correct or not. For example, this is invalid code:
```mlir
%tmp = call @S1(%X, %0, %1)
domain: (10 <= %i < %N), (0 <= %j < %N)
schedule: (i, j)
call @S2(%tmp, %A, %0, %1)
domain: (0 <= %i < %N), (0 <= %j < %N)
schedule: (i, j)
```
Because `%tmp` isn't defined on some iterations of the %i loop.
This matters because it makes the verifier more complicated, but more
significantly, it means that load promotion and other optimizations that will
produce SSA form will need to be aware of this and be able to model what codegen
does.
An emergent property of this that we discussed recently is that PHI nodes in
mlfunc's (if we support them) will also have to have domains.
### Lack of redundancy in IR
The traditional form has multiple encodings for the same sorts of behavior: you
end up having bits on `affine.for` loops to specify whether codegen should use
"atomic/separate" policies, unroll loops, etc. Instructions can be split or can
generate multiple copies of their instruction because of overlapping domains,
etc.
This is a problem for analyses and cost models, because they each have to reason
about these additional forms in the IR.
### Suitability to purpose: lowering to machine code
One of the main drivers for this work is lowering to low-level accelerator code,
including two-dimensional vectorization, insertion of DMAs, and other
utilization of the matrix accelerator units. In the author's opinion, the extra
compactness of the traditional form is a negative for this purpose: reasoning
about the generated machine code will require understanding the mapping from
mlfunc to lowered code, which means that it must understand what code generation
will do.
In the simplified form, the effect of "code generation" is always obvious from
the IR itself, which should make it easier to perform vectorization to target
instructions and other analyses we need to perform.
## Third Alternative: two different levels of mlfunc
One hybrid alternative is to support both the traditional and simplified forms
of mlfunc in our IR.
The stages could look like this, for example:
1. Early performance transformations could be done on the traditional form.
1. Partial code generation lowers to the simplified form
1. Target specific lowering phases for tiling, and vectorization and other 2D
transforms that don't benefit much from the traditional form could be run.
1. Final codegen to a cfg func can be done when all of the instructions are
replaced with ones valid on the target.
While this is possible, it isn't clear what would justify the complexity of this
approach. Unless there is a super compelling reason for this, it would be nice
to not do this. **Update:** we discussed this as a design team and agreed that
this wouldn't be a good way to go.
| {
"pile_set_name": "Github"
} |
/* @flow */
import { dirRE, onRE } from './parser/index'
// these keywords should not appear inside expressions, but operators like
// typeof, instanceof and in are allowed
const prohibitedKeywordRE = new RegExp('\\b' + (
'do,if,for,let,new,try,var,case,else,with,await,break,catch,class,const,' +
'super,throw,while,yield,delete,export,import,return,switch,default,' +
'extends,finally,continue,debugger,function,arguments'
).split(',').join('\\b|\\b') + '\\b')
// these unary operators should not be used as property/method names
const unaryOperatorsRE = new RegExp('\\b' + (
'delete,typeof,void'
).split(',').join('\\s*\\([^\\)]*\\)|\\b') + '\\s*\\([^\\)]*\\)')
// check valid identifier for v-for
const identRE = /[A-Za-z_$][\w$]*/
// strip strings in expressions
const stripStringRE = /'(?:[^'\\]|\\.)*'|"(?:[^"\\]|\\.)*"|`(?:[^`\\]|\\.)*\$\{|\}(?:[^`\\]|\\.)*`|`(?:[^`\\]|\\.)*`/g
// detect problematic expressions in a template
export function detectErrors (ast: ?ASTNode): Array<string> {
const errors: Array<string> = []
if (ast) {
checkNode(ast, errors)
}
return errors
}
function checkNode (node: ASTNode, errors: Array<string>) {
if (node.type === 1) {
for (const name in node.attrsMap) {
if (dirRE.test(name)) {
const value = node.attrsMap[name]
if (value) {
if (name === 'v-for') {
checkFor(node, `v-for="${value}"`, errors)
} else if (onRE.test(name)) {
checkEvent(value, `${name}="${value}"`, errors)
} else {
checkExpression(value, `${name}="${value}"`, errors)
}
}
}
}
if (node.children) {
for (let i = 0; i < node.children.length; i++) {
checkNode(node.children[i], errors)
}
}
} else if (node.type === 2) {
checkExpression(node.expression, node.text, errors)
}
}
function checkEvent (exp: string, text: string, errors: Array<string>) {
const keywordMatch = exp.replace(stripStringRE, '').match(unaryOperatorsRE)
if (keywordMatch) {
errors.push(
`avoid using JavaScript unary operator as property name: ` +
`"${keywordMatch[0]}" in expression ${text.trim()}`
)
}
checkExpression(exp, text, errors)
}
function checkFor (node: ASTElement, text: string, errors: Array<string>) {
checkExpression(node.for || '', text, errors)
checkIdentifier(node.alias, 'v-for alias', text, errors)
checkIdentifier(node.iterator1, 'v-for iterator', text, errors)
checkIdentifier(node.iterator2, 'v-for iterator', text, errors)
}
function checkIdentifier (ident: ?string, type: string, text: string, errors: Array<string>) {
if (typeof ident === 'string' && !identRE.test(ident)) {
errors.push(`invalid ${type} "${ident}" in expression: ${text.trim()}`)
}
}
function checkExpression (exp: string, text: string, errors: Array<string>) {
try {
new Function(`return ${exp}`)
} catch (e) {
const keywordMatch = exp.replace(stripStringRE, '').match(prohibitedKeywordRE)
if (keywordMatch) {
errors.push(
`avoid using JavaScript keyword as property name: ` +
`"${keywordMatch[0]}" in expression ${text.trim()}`
)
} else {
errors.push(`invalid expression: ${text.trim()}`)
}
}
}
| {
"pile_set_name": "Github"
} |
#!/bin/bash
# Simple test script to ping local endpoint
curl -X GET \
http://localhost:5000/users
curl -X GET \
http://localhost:5000/tracks
| {
"pile_set_name": "Github"
} |
// Tencent is pleased to support the open source community by making RapidJSON available.
//
// Copyright (C) 2015 THL A29 Limited, a Tencent company, and Milo Yip. All rights reserved.
//
// Licensed under the MIT License (the "License"); you may not use this file except
// in compliance with the License. You may obtain a copy of the License at
//
// http://opensource.org/licenses/MIT
//
// Unless required by applicable law or agreed to in writing, software distributed
// under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
// CONDITIONS OF ANY KIND, either express or implied. See the License for the
// specific language governing permissions and limitations under the License.
#ifndef RAPIDJSON_MEMORYSTREAM_H_
#define RAPIDJSON_MEMORYSTREAM_H_
#include "stream.h"
#ifdef __clang__
RAPIDJSON_DIAG_PUSH
RAPIDJSON_DIAG_OFF(unreachable-code)
RAPIDJSON_DIAG_OFF(missing-noreturn)
#endif
RAPIDJSON_NAMESPACE_BEGIN
//! Represents an in-memory input byte stream.
/*!
This class is mainly for being wrapped by EncodedInputStream or AutoUTFInputStream.
It is similar to FileReadBuffer but the source is an in-memory buffer instead of a file.
Differences between MemoryStream and StringStream:
1. StringStream has encoding but MemoryStream is a byte stream.
2. MemoryStream needs size of the source buffer and the buffer don't need to be null terminated. StringStream assume null-terminated string as source.
3. MemoryStream supports Peek4() for encoding detection. StringStream is specified with an encoding so it should not have Peek4().
\note implements Stream concept
*/
struct MemoryStream {
typedef char Ch; // byte
MemoryStream(const Ch *src, size_t size) : src_(src), begin_(src), end_(src + size), size_(size) {}
Ch Peek() const { return RAPIDJSON_UNLIKELY(src_ == end_) ? '\0' : *src_; }
Ch Take() { return RAPIDJSON_UNLIKELY(src_ == end_) ? '\0' : *src_++; }
size_t Tell() const { return static_cast<size_t>(src_ - begin_); }
Ch* PutBegin() { RAPIDJSON_ASSERT(false); return 0; }
void Put(Ch) { RAPIDJSON_ASSERT(false); }
void Flush() { RAPIDJSON_ASSERT(false); }
size_t PutEnd(Ch*) { RAPIDJSON_ASSERT(false); return 0; }
// For encoding detection only.
const Ch* Peek4() const {
return Tell() + 4 <= size_ ? src_ : 0;
}
const Ch* src_; //!< Current read position.
const Ch* begin_; //!< Original head of the string.
const Ch* end_; //!< End of stream.
size_t size_; //!< Size of the stream.
};
RAPIDJSON_NAMESPACE_END
#ifdef __clang__
RAPIDJSON_DIAG_POP
#endif
#endif // RAPIDJSON_MEMORYBUFFER_H_
| {
"pile_set_name": "Github"
} |
#ifndef BOOST_ARCHIVE_ITERATORS_UNESCAPE_HPP
#define BOOST_ARCHIVE_ITERATORS_UNESCAPE_HPP
// MS compatible compilers support #pragma once
#if defined(_MSC_VER)
# pragma once
#endif
/////////1/////////2/////////3/////////4/////////5/////////6/////////7/////////8
// unescape.hpp
// (C) Copyright 2002 Robert Ramey - http://www.rrsd.com .
// Use, modification and distribution is subject to the Boost Software
// License, Version 1.0. (See accompanying file LICENSE_1_0.txt or copy at
// http://www.boost.org/LICENSE_1_0.txt)
// See http://www.boost.org for updates, documentation, and revision history.
#include <boost/assert.hpp>
#include <boost/iterator/iterator_adaptor.hpp>
#include <boost/pointee.hpp>
namespace boost {
namespace archive {
namespace iterators {
/////////1/////////2/////////3/////////4/////////5/////////6/////////7/////////8
// class used by text archives to translate char strings to wchar_t
// strings of the currently selected locale
template<class Derived, class Base>
class unescape
: public boost::iterator_adaptor<
unescape<Derived, Base>,
Base,
typename pointee<Base>::type,
single_pass_traversal_tag,
typename pointee<Base>::type
>
{
friend class boost::iterator_core_access;
typedef typename boost::iterator_adaptor<
unescape<Derived, Base>,
Base,
typename pointee<Base>::type,
single_pass_traversal_tag,
typename pointee<Base>::type
> super_t;
typedef unescape<Derived, Base> this_t;
public:
typedef typename this_t::value_type value_type;
typedef typename this_t::reference reference;
private:
value_type dereference_impl() {
if(! m_full){
m_current_value = static_cast<Derived *>(this)->drain();
m_full = true;
}
return m_current_value;
}
reference dereference() const {
return const_cast<this_t *>(this)->dereference_impl();
}
value_type m_current_value;
bool m_full;
void increment(){
++(this->base_reference());
dereference_impl();
m_full = false;
};
public:
unescape(Base base) :
super_t(base),
m_full(false)
{}
};
} // namespace iterators
} // namespace archive
} // namespace boost
#endif // BOOST_ARCHIVE_ITERATORS_UNESCAPE_HPP
| {
"pile_set_name": "Github"
} |
/*
* Copyright 2015 The WebRTC Project Authors. All rights reserved.
*
* Use of this source code is governed by a BSD-style license
* that can be found in the LICENSE file in the root of the source
* tree. An additional intellectual property rights grant can be found
* in the file PATENTS. All contributing project authors may
* be found in the AUTHORS file in the root of the source tree.
*/
#include "rtc_base/buffer_queue.h"
#include <string.h>
#include "test/gtest.h"
namespace rtc {
TEST(BufferQueueTest, TestAll) {
const size_t kSize = 16;
const char in[kSize * 2 + 1] = "0123456789ABCDEFGHIJKLMNOPQRSTUV";
char out[kSize * 2];
size_t bytes;
BufferQueue queue1(1, kSize);
BufferQueue queue2(2, kSize);
// The queue is initially empty.
EXPECT_EQ(0u, queue1.size());
EXPECT_FALSE(queue1.ReadFront(out, kSize, &bytes));
// A write should succeed.
EXPECT_TRUE(queue1.WriteBack(in, kSize, &bytes));
EXPECT_EQ(kSize, bytes);
EXPECT_EQ(1u, queue1.size());
// The queue is full now (only one buffer allowed).
EXPECT_FALSE(queue1.WriteBack(in, kSize, &bytes));
EXPECT_EQ(1u, queue1.size());
// Reading previously written buffer.
EXPECT_TRUE(queue1.ReadFront(out, kSize, &bytes));
EXPECT_EQ(kSize, bytes);
EXPECT_EQ(0, memcmp(in, out, kSize));
// The queue is empty again now.
EXPECT_FALSE(queue1.ReadFront(out, kSize, &bytes));
EXPECT_EQ(0u, queue1.size());
// Reading only returns available data.
EXPECT_TRUE(queue1.WriteBack(in, kSize, &bytes));
EXPECT_EQ(kSize, bytes);
EXPECT_EQ(1u, queue1.size());
EXPECT_TRUE(queue1.ReadFront(out, kSize * 2, &bytes));
EXPECT_EQ(kSize, bytes);
EXPECT_EQ(0, memcmp(in, out, kSize));
EXPECT_EQ(0u, queue1.size());
// Reading maintains buffer boundaries.
EXPECT_TRUE(queue2.WriteBack(in, kSize / 2, &bytes));
EXPECT_EQ(1u, queue2.size());
EXPECT_TRUE(queue2.WriteBack(in + kSize / 2, kSize / 2, &bytes));
EXPECT_EQ(2u, queue2.size());
EXPECT_TRUE(queue2.ReadFront(out, kSize, &bytes));
EXPECT_EQ(kSize / 2, bytes);
EXPECT_EQ(0, memcmp(in, out, kSize / 2));
EXPECT_EQ(1u, queue2.size());
EXPECT_TRUE(queue2.ReadFront(out, kSize, &bytes));
EXPECT_EQ(kSize / 2, bytes);
EXPECT_EQ(0, memcmp(in + kSize / 2, out, kSize / 2));
EXPECT_EQ(0u, queue2.size());
// Reading truncates buffers.
EXPECT_TRUE(queue2.WriteBack(in, kSize / 2, &bytes));
EXPECT_EQ(1u, queue2.size());
EXPECT_TRUE(queue2.WriteBack(in + kSize / 2, kSize / 2, &bytes));
EXPECT_EQ(2u, queue2.size());
// Read first packet partially in too-small buffer.
EXPECT_TRUE(queue2.ReadFront(out, kSize / 4, &bytes));
EXPECT_EQ(kSize / 4, bytes);
EXPECT_EQ(0, memcmp(in, out, kSize / 4));
EXPECT_EQ(1u, queue2.size());
// Remainder of first packet is truncated, reading starts with next packet.
EXPECT_TRUE(queue2.ReadFront(out, kSize, &bytes));
EXPECT_EQ(kSize / 2, bytes);
EXPECT_EQ(0, memcmp(in + kSize / 2, out, kSize / 2));
EXPECT_EQ(0u, queue2.size());
}
} // namespace rtc
| {
"pile_set_name": "Github"
} |
// This is a part of the Microsoft Foundation Classes C++ library.
// Copyright (C) 1992-1998 Microsoft Corporation
// All rights reserved.
//
// This source code is only intended as a supplement to the
// Microsoft Foundation Classes Reference and related
// electronic documentation provided with the library.
// See these sources for detailed information regarding the
// Microsoft Foundation Classes product.
#include "stdafx.h"
#ifdef _DEBUG
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
#define new DEBUG_NEW
/////////////////////////////////////////////////////////////////////////////
// CSemaphore
CSemaphore::CSemaphore(LONG lInitialCount, LONG lMaxCount,
LPCTSTR pstrName, LPSECURITY_ATTRIBUTES lpsaAttributes)
: CSyncObject(pstrName)
{
ASSERT(lMaxCount > 0);
ASSERT(lInitialCount <= lMaxCount);
m_hObject = ::CreateSemaphore(lpsaAttributes, lInitialCount, lMaxCount,
pstrName);
if (m_hObject == NULL)
AfxThrowResourceException();
}
CSemaphore::~CSemaphore()
{
}
BOOL CSemaphore::Unlock(LONG lCount, LPLONG lpPrevCount /* =NULL */)
{
return ::ReleaseSemaphore(m_hObject, lCount, lpPrevCount);
}
/////////////////////////////////////////////////////////////////////////////
// CMutex
CMutex::CMutex(BOOL bInitiallyOwn, LPCTSTR pstrName,
LPSECURITY_ATTRIBUTES lpsaAttribute /* = NULL */)
: CSyncObject(pstrName)
{
m_hObject = ::CreateMutex(lpsaAttribute, bInitiallyOwn, pstrName);
if (m_hObject == NULL)
AfxThrowResourceException();
}
CMutex::~CMutex()
{
}
BOOL CMutex::Unlock()
{
return ::ReleaseMutex(m_hObject);
}
/////////////////////////////////////////////////////////////////////////////
// CEvent
CEvent::CEvent(BOOL bInitiallyOwn, BOOL bManualReset, LPCTSTR pstrName,
LPSECURITY_ATTRIBUTES lpsaAttribute)
: CSyncObject(pstrName)
{
m_hObject = ::CreateEvent(lpsaAttribute, bManualReset,
bInitiallyOwn, pstrName);
if (m_hObject == NULL)
AfxThrowResourceException();
}
CEvent::~CEvent()
{
}
BOOL CEvent::Unlock()
{
return TRUE;
}
/////////////////////////////////////////////////////////////////////////////
// CSingleLock
CSingleLock::CSingleLock(CSyncObject* pObject, BOOL bInitialLock)
{
ASSERT(pObject != NULL);
ASSERT(pObject->IsKindOf(RUNTIME_CLASS(CSyncObject)));
m_pObject = pObject;
m_hObject = pObject->m_hObject;
m_bAcquired = FALSE;
if (bInitialLock)
Lock();
}
BOOL CSingleLock::Lock(DWORD dwTimeOut /* = INFINITE */)
{
ASSERT(m_pObject != NULL || m_hObject != NULL);
ASSERT(!m_bAcquired);
m_bAcquired = m_pObject->Lock(dwTimeOut);
return m_bAcquired;
}
BOOL CSingleLock::Unlock()
{
ASSERT(m_pObject != NULL);
if (m_bAcquired)
m_bAcquired = !m_pObject->Unlock();
// successfully unlocking means it isn't acquired
return !m_bAcquired;
}
BOOL CSingleLock::Unlock(LONG lCount, LPLONG lpPrevCount /* = NULL */)
{
ASSERT(m_pObject != NULL);
if (m_bAcquired)
m_bAcquired = !m_pObject->Unlock(lCount, lpPrevCount);
// successfully unlocking means it isn't acquired
return !m_bAcquired;
}
/////////////////////////////////////////////////////////////////////////////
// CMultiLock
CMultiLock::CMultiLock(CSyncObject* pObjects[], DWORD dwCount,
BOOL bInitialLock)
{
ASSERT(dwCount > 0 && dwCount <= MAXIMUM_WAIT_OBJECTS);
ASSERT(pObjects != NULL);
m_ppObjectArray = pObjects;
m_dwCount = dwCount;
// as an optimization, skip allocating array if
// we can use a small, preallocated bunch of handles
if (m_dwCount > _countof(m_hPreallocated))
{
m_pHandleArray = new HANDLE[m_dwCount];
m_bLockedArray = new BOOL[m_dwCount];
}
else
{
m_pHandleArray = m_hPreallocated;
m_bLockedArray = m_bPreallocated;
}
// get list of handles from array of objects passed
for (DWORD i = 0; i <m_dwCount; i++)
{
ASSERT_VALID(pObjects[i]);
ASSERT(pObjects[i]->IsKindOf(RUNTIME_CLASS(CSyncObject)));
// can't wait for critical sections
ASSERT(!pObjects[i]->IsKindOf(RUNTIME_CLASS(CCriticalSection)));
m_pHandleArray[i] = pObjects[i]->m_hObject;
m_bLockedArray[i] = FALSE;
}
if (bInitialLock)
Lock();
}
CMultiLock::~CMultiLock()
{
Unlock();
if (m_pHandleArray != m_hPreallocated)
{
delete[] m_bLockedArray;
delete[] m_pHandleArray;
}
}
DWORD CMultiLock::Lock(DWORD dwTimeOut /* = INFINITE */,
BOOL bWaitForAll /* = TRUE */, DWORD dwWakeMask /* = 0 */)
{
DWORD dwResult;
if (dwWakeMask == 0)
dwResult = ::WaitForMultipleObjects(m_dwCount,
m_pHandleArray, bWaitForAll, dwTimeOut);
else
dwResult = ::MsgWaitForMultipleObjects(m_dwCount,
m_pHandleArray, bWaitForAll, dwTimeOut, dwWakeMask);
if (dwResult >= WAIT_OBJECT_0 && dwResult < (WAIT_OBJECT_0 + m_dwCount))
{
if (bWaitForAll)
{
for (DWORD i = 0; i < m_dwCount; i++)
m_bLockedArray[i] = TRUE;
}
else
{
ASSERT((dwResult - WAIT_OBJECT_0) >= 0);
m_bLockedArray[dwResult - WAIT_OBJECT_0] = TRUE;
}
}
return dwResult;
}
BOOL CMultiLock::Unlock()
{
for (DWORD i=0; i < m_dwCount; i++)
{
if (m_bLockedArray[i])
m_bLockedArray[i] = !m_ppObjectArray[i]->Unlock();
}
return TRUE;
}
BOOL CMultiLock::Unlock(LONG lCount, LPLONG lpPrevCount /* =NULL */)
{
BOOL bGotOne = FALSE;
for (DWORD i=0; i < m_dwCount; i++)
{
if (m_bLockedArray[i])
{
CSemaphore* pSemaphore = STATIC_DOWNCAST(CSemaphore, m_ppObjectArray[i]);
if (pSemaphore != NULL)
{
bGotOne = TRUE;
m_bLockedArray[i] = !m_ppObjectArray[i]->Unlock(lCount, lpPrevCount);
}
}
}
return bGotOne;
}
#ifdef AFX_INIT_SEG
#pragma code_seg(AFX_INIT_SEG)
#endif
IMPLEMENT_DYNAMIC(CEvent, CSyncObject)
IMPLEMENT_DYNAMIC(CSemaphore, CSyncObject)
IMPLEMENT_DYNAMIC(CMutex, CSyncObject)
/////////////////////////////////////////////////////////////////////////////
| {
"pile_set_name": "Github"
} |
# This file is part of BlackArch Linux ( https://www.blackarch.org/ ).
# See COPYING for license details.
pkgname=blackarch-config-x11
pkgver=21.e38cf84
pkgrel=1
pkgdesc='BlackArch config for the X11 user settings.'
arch=('any')
groups=('blackarch-config')
url='https://github.com/BlackArch/blackarch-config-x11'
license=('custom:unknown')
depends=('xterm' 'rxvt-unicode' 'terminus-font')
makedepends=('git')
source=("git+https://github.com/BlackArch/$pkgname.git")
sha512sums=('SKIP')
install="$pkgname.install"
pkgver() {
cd $pkgname
echo $(git rev-list --count HEAD).$(git rev-parse --short HEAD)
}
package() {
cd $pkgname
install -dm 755 "$pkgdir/usr/share/blackarch/config/x11"
cp -a * "$pkgdir/usr/share/blackarch/config/x11/"
}
| {
"pile_set_name": "Github"
} |
<mods xmlns="http://www.loc.gov/mods/v3" xmlns:exslt="http://exslt.org/common" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xlink="http://www.w3.org/1999/xlink" xsi:schemaLocation="http://www.loc.gov/mods/v3 http://www.loc.gov/standards/mods/v3/mods-3-3.xsd" version="3.3" ID="P0b002ee181288845">
<name type="corporate">
<namePart>United States Government Printing Office</namePart>
<role>
<roleTerm type="text" authority="marcrelator">printer</roleTerm>
<roleTerm type="code" authority="marcrelator">prt</roleTerm>
</role>
<role>
<roleTerm type="text" authority="marcrelator">distributor</roleTerm>
<roleTerm type="code" authority="marcrelator">dst</roleTerm>
</role>
</name>
<name type="corporate">
<namePart>United States</namePart>
<namePart>United States District Court Middle District of Georgia</namePart>
<role>
<roleTerm type="text" authority="marcrelator">author</roleTerm>
<roleTerm type="code" authority="marcrelator">aut</roleTerm>
</role>
<description>Government Organization</description>
</name>
<typeOfResource>text</typeOfResource>
<genre authority="marcgt">government publication</genre>
<language>
<languageTerm authority="iso639-2b" type="code">eng</languageTerm>
</language>
<extension>
<collectionCode>USCOURTS</collectionCode>
<category>Judicial Publications</category>
<branch>judicial</branch>
<dateIngested>2011-12-19</dateIngested>
</extension>
<originInfo>
<publisher>Administrative Office of the United States Courts</publisher>
<dateIssued encoding="w3cdtf">2006-01-04</dateIssued>
<issuance>monographic</issuance>
</originInfo>
<physicalDescription>
<note type="source content type">deposited</note>
<digitalOrigin>born digital</digitalOrigin>
</physicalDescription>
<classification authority="sudocs">JU 4.15</classification>
<identifier type="uri">http://www.gpo.gov/fdsys/pkg/USCOURTS-gamd-5_05-cv-00214</identifier>
<identifier type="local">P0b002ee181288845</identifier>
<recordInfo>
<recordContentSource authority="marcorg">DGPO</recordContentSource>
<recordCreationDate encoding="w3cdtf">2011-12-19</recordCreationDate>
<recordChangeDate encoding="w3cdtf">2011-12-19</recordChangeDate>
<recordIdentifier source="DGPO">USCOURTS-gamd-5_05-cv-00214</recordIdentifier>
<recordOrigin>machine generated</recordOrigin>
<languageOfCataloging>
<languageTerm authority="iso639-2b" type="code">eng</languageTerm>
</languageOfCataloging>
</recordInfo>
<accessCondition type="GPO scope determination">fdlp</accessCondition>
<extension>
<docClass>USCOURTS</docClass>
<accessId>USCOURTS-gamd-5_05-cv-00214</accessId>
<courtType>District</courtType>
<courtCode>gamd</courtCode>
<courtCircuit>11th</courtCircuit>
<courtState>Georgia</courtState>
<courtSortOrder>2125</courtSortOrder>
<caseNumber>5:05-cv-00214</caseNumber>
<caseOffice>Macon</caseOffice>
<caseType>civil</caseType>
<natureSuitCode>790</natureSuitCode>
<natureSuit>Other Labor Litigation</natureSuit>
<cause>29:206 Collect Unpaid Wages</cause>
<party fullName="CACI International, Inc." lastName="CACI International, Inc." role="Defendant"></party>
<party firstName="Vicky" fullName="Vicky Thomason" lastName="Thomason" role="Plaintiff"></party>
</extension>
<titleInfo>
<title>Thomason v. CACI International, Inc.</title>
<partNumber>5:05-cv-00214</partNumber>
</titleInfo>
<location>
<url access="object in context" displayLabel="Content Detail">http://www.gpo.gov/fdsys/pkg/USCOURTS-gamd-5_05-cv-00214/content-detail.html</url>
</location>
<classification authority="sudocs">JU 4.15</classification>
<identifier type="preferred citation">5:05-cv-00214;05-214</identifier>
<name type="corporate">
<namePart>United States District Court Middle District of Georgia</namePart>
<namePart>11th Circuit</namePart>
<namePart>Macon</namePart>
<affiliation>U.S. Courts</affiliation>
<role>
<roleTerm authority="marcrelator" type="text">author</roleTerm>
<roleTerm authority="marcrelator" type="code">aut</roleTerm>
</role>
</name>
<name type="personal">
<displayForm>CACI International, Inc.</displayForm>
<namePart type="family">CACI International, Inc.</namePart>
<namePart type="given"></namePart>
<namePart type="termsOfAddress"></namePart>
<description>Defendant</description>
</name>
<name type="personal">
<displayForm>Vicky Thomason</displayForm>
<namePart type="family">Thomason</namePart>
<namePart type="given">Vicky</namePart>
<namePart type="termsOfAddress"></namePart>
<description>Plaintiff</description>
</name>
<extension>
<docClass>USCOURTS</docClass>
<accessId>USCOURTS-gamd-5_05-cv-00214</accessId>
<courtType>District</courtType>
<courtCode>gamd</courtCode>
<courtCircuit>11th</courtCircuit>
<courtState>Georgia</courtState>
<courtSortOrder>2125</courtSortOrder>
<caseNumber>5:05-cv-00214</caseNumber>
<caseOffice>Macon</caseOffice>
<caseType>civil</caseType>
<natureSuitCode>790</natureSuitCode>
<natureSuit>Other Labor Litigation</natureSuit>
<cause>29:206 Collect Unpaid Wages</cause>
<party fullName="CACI International, Inc." lastName="CACI International, Inc." role="Defendant"></party>
<party firstName="Vicky" fullName="Vicky Thomason" lastName="Thomason" role="Plaintiff"></party>
<state>Georgia</state>
</extension>
<relatedItem type="constituent" ID="id-USCOURTS-gamd-5_05-cv-00214-0" xlink:href="http://www.gpo.gov/fdsys/granule/USCOURTS-gamd-5_05-cv-00214/USCOURTS-gamd-5_05-cv-00214-0/mods.xml">
<titleInfo>
<title>Thomason v. CACI International, Inc.</title>
<subTitle>ORDER granting 3 Motion to Dismiss and ordering mediation and/or arbitration. Signed by Judge Wilbur D. Owens Jr. on 1/3/2006. (cf)</subTitle>
<partNumber>0</partNumber>
</titleInfo>
<originInfo>
<dateIssued>2006-01-04</dateIssued>
</originInfo>
<relatedItem xlink:href="http://www.gpo.gov/fdsys/pkg/pdf/USCOURTS-gamd-5_05-cv-00214-0.pdf" type="otherFormat">
<identifier type="FDsys Unique ID">D09002ee1812ac883</identifier>
</relatedItem>
<identifier type="uri">http://www.gpo.gov/fdsys/granule/USCOURTS-gamd-5_05-cv-00214/USCOURTS-gamd-5_05-cv-00214-0</identifier>
<identifier type="former granule identifier">gamd-5_05-cv-00214_0.pdf</identifier>
<location>
<url access="object in context" displayLabel="Content Detail">http://www.gpo.gov/fdsys/granule/USCOURTS-gamd-5_05-cv-00214/USCOURTS-gamd-5_05-cv-00214-0/content-detail.html</url>
<url access="raw object" displayLabel="PDF rendition">http://www.gpo.gov/fdsys/pkg/USCOURTS-gamd-5_05-cv-00214/pdf/USCOURTS-gamd-5_05-cv-00214-0.pdf</url>
</location>
<extension>
<searchTitle>USCOURTS 5:05-cv-00214; Thomason v. CACI International, Inc.; </searchTitle>
<courtName>United States District Court Middle District of Georgia</courtName>
<state>Georgia</state>
<accessId>USCOURTS-gamd-5_05-cv-00214-0</accessId>
<sequenceNumber>0</sequenceNumber>
<dateIssued>2006-01-04</dateIssued>
<docketText>ORDER granting 3 Motion to Dismiss and ordering mediation and/or arbitration. Signed by Judge Wilbur D. Owens Jr. on 1/3/2006. (cf)</docketText>
</extension>
</relatedItem>
</mods> | {
"pile_set_name": "Github"
} |
using System;
using UnityEditor;
using UnityEngine;
namespace Chisel.Components
{
public struct GameObjectState
{
public int layer;
#if UNITY_EDITOR
public UnityEditor.StaticEditorFlags staticFlags;
#endif
public static GameObjectState Create(GameObject modelGameObject)
{
return new GameObjectState
{
layer = modelGameObject.layer,
#if UNITY_EDITOR
staticFlags = UnityEditor.GameObjectUtility.GetStaticEditorFlags(modelGameObject)
#endif
};
}
}
//TODO: Move this somewhere else
public static class ChiselObjectUtility
{
public static void SafeDestroy(UnityEngine.Object obj)
{
if (!obj)
return;
obj.hideFlags = UnityEngine.HideFlags.None;
#if UNITY_EDITOR
if (!UnityEditor.EditorApplication.isPlaying)
UnityEngine.Object.DestroyImmediate(obj);
else
#endif
UnityEngine.Object.Destroy(obj);
}
public static void SafeDestroyWithUndo(UnityEngine.Object obj)
{
if (!obj)
return;
#if UNITY_EDITOR
if (!UnityEditor.EditorApplication.isPlaying)
{
UnityEditor.Undo.RecordObject(obj, "Destroying object");
obj.hideFlags = UnityEngine.HideFlags.None;
UnityEditor.Undo.DestroyObjectImmediate(obj);
} else
#endif
{
Debug.Log("Undo not possible");
obj.hideFlags = UnityEngine.HideFlags.None;
UnityEngine.Object.Destroy(obj);
}
}
public static void SafeDestroy(UnityEngine.GameObject obj, bool ignoreHierarchyEvents = false)
{
if (!obj)
return;
if (ignoreHierarchyEvents)
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = true;
try
{
obj.hideFlags = UnityEngine.HideFlags.None;
obj.transform.hideFlags = HideFlags.None;
#if UNITY_EDITOR
if (!UnityEditor.EditorApplication.isPlaying)
UnityEngine.Object.DestroyImmediate(obj);
else
#endif
UnityEngine.Object.Destroy(obj);
}
finally
{
if (ignoreHierarchyEvents)
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = false;
}
}
public static void SafeDestroyWithUndo(UnityEngine.GameObject obj, bool ignoreHierarchyEvents = false)
{
if (!obj)
return;
if (ignoreHierarchyEvents)
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = true;
try
{
#if UNITY_EDITOR
if (!UnityEditor.EditorApplication.isPlaying)
{
UnityEditor.Undo.RecordObjects(new UnityEngine.Object[] { obj, obj.transform }, "Destroying object");
obj.hideFlags = UnityEngine.HideFlags.None;
obj.transform.hideFlags = HideFlags.None;
UnityEditor.Undo.DestroyObjectImmediate(obj);
} else
#endif
{
Debug.Log("Undo not possible");
obj.hideFlags = UnityEngine.HideFlags.None;
obj.transform.hideFlags = HideFlags.None;
UnityEngine.Object.Destroy(obj);
}
}
finally
{
if (ignoreHierarchyEvents)
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = false;
}
}
public static void ResetTransform(Transform transform)
{
var prevLocalPosition = transform.localPosition;
var prevLocalRotation = transform.localRotation;
var prevLocalScale = transform.localScale;
if (prevLocalPosition.x != 0 ||
prevLocalPosition.y != 0 ||
prevLocalPosition.z != 0)
transform.localPosition = Vector3.zero;
if (prevLocalRotation != Quaternion.identity)
transform.localRotation = Quaternion.identity;
if (prevLocalScale.x != 1 ||
prevLocalScale.y != 1 ||
prevLocalScale.z != 1)
transform.localScale = Vector3.one;
}
public static void ResetTransform(Transform transform, Transform requiredParent)
{
if (transform.parent != requiredParent)
transform.SetParent(requiredParent, false);
ResetTransform(transform);
}
public static GameObject CreateGameObject(string name, Transform parent, params Type[] components)
{
var parentScene = parent.gameObject.scene;
var oldActiveScene = UnityEngine.SceneManagement.SceneManager.GetActiveScene();
if (parentScene != oldActiveScene)
UnityEngine.SceneManagement.SceneManager.SetActiveScene(parentScene);
try
{
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = true;
var newGameObject = new GameObject(name, components);
newGameObject.SetActive(false);
try
{
var transform = newGameObject.GetComponent<Transform>();
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = true;
transform.SetParent(parent, false);
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = false;
ResetTransform(transform);
}
finally
{
newGameObject.SetActive(true);
}
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = false;
return newGameObject;
}
finally
{
if (parentScene != oldActiveScene)
UnityEngine.SceneManagement.SceneManager.SetActiveScene(oldActiveScene);
}
}
public static GameObject CreateGameObject(string name, Transform parent)
{
var parentScene = parent.gameObject.scene;
var oldActiveScene = UnityEngine.SceneManagement.SceneManager.GetActiveScene();
if (parentScene != oldActiveScene)
UnityEngine.SceneManagement.SceneManager.SetActiveScene(parentScene);
try
{
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = true;
var newGameObject = new GameObject(name);
newGameObject.SetActive(false);
try
{
var transform = newGameObject.GetComponent<Transform>();
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = true;
transform.SetParent(parent, false);
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = false;
ResetTransform(transform);
}
finally
{
newGameObject.SetActive(true);
}
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = false;
return newGameObject;
}
finally
{
if (parentScene != oldActiveScene)
UnityEngine.SceneManagement.SceneManager.SetActiveScene(oldActiveScene);
}
}
public static GameObject CreateGameObject(string name, Transform parent, GameObjectState state, bool debugHelperRenderer = false)
{
var parentScene = parent.gameObject.scene;
var oldActiveScene = UnityEngine.SceneManagement.SceneManager.GetActiveScene();
if (parentScene != oldActiveScene)
UnityEngine.SceneManagement.SceneManager.SetActiveScene(parentScene);
try
{
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = true;
var newGameObject = new GameObject(name);
newGameObject.SetActive(false);
try
{
UpdateContainerFlags(newGameObject, state, debugHelperRenderer: debugHelperRenderer);
var transform = newGameObject.GetComponent<Transform>();
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = true;
transform.SetParent(parent, false);
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = false;
ResetTransform(transform);
}
finally
{
newGameObject.SetActive(true);
}
ChiselNodeHierarchyManager.ignoreNextChildrenChanged = false;
return newGameObject;
}
finally
{
if (parentScene != oldActiveScene)
UnityEngine.SceneManagement.SceneManager.SetActiveScene(oldActiveScene);
}
}
const HideFlags kGameObjectHideFlags = HideFlags.NotEditable;
const HideFlags kEditorGameObjectHideFlags = kGameObjectHideFlags | HideFlags.DontSaveInBuild;
const HideFlags kTransformHideFlags = HideFlags.NotEditable;// | HideFlags.HideInInspector;
const HideFlags kComponentHideFlags = HideFlags.HideInHierarchy | HideFlags.NotEditable; // Avoids MeshCollider showing wireframe
internal static void UpdateContainerFlags(GameObject gameObject, GameObjectState state, bool debugHelperRenderer = false)
{
var transform = gameObject.transform;
var desiredGameObjectFlags = debugHelperRenderer ? kEditorGameObjectHideFlags : kGameObjectHideFlags;
var desiredLayer = debugHelperRenderer ? 0 : state.layer;
if (gameObject.layer != desiredLayer ) gameObject.layer = desiredLayer;
if (gameObject.hideFlags != desiredGameObjectFlags) gameObject.hideFlags = desiredGameObjectFlags;
if (transform .hideFlags != kTransformHideFlags ) transform .hideFlags = kTransformHideFlags;
#if UNITY_EDITOR
StaticEditorFlags desiredStaticFlags;
if (debugHelperRenderer)
{
desiredStaticFlags = (StaticEditorFlags)0;
} else
{
desiredStaticFlags = UnityEditor.GameObjectUtility.GetStaticEditorFlags(gameObject);
}
if (desiredStaticFlags != state.staticFlags)
UnityEditor.GameObjectUtility.SetStaticEditorFlags(gameObject, desiredStaticFlags);
#endif
}
internal static void UpdateContainerFlags(Component component, GameObjectState modelState)
{
var gameObject = component.gameObject;
UpdateContainerFlags(gameObject, modelState);
if (component.hideFlags != kComponentHideFlags ) component.hideFlags = kComponentHideFlags;
}
public static void RemoveContainerFlags(GameObject gameObject)
{
var transform = (gameObject) ? gameObject.transform : null;
if (gameObject) gameObject.hideFlags = HideFlags.None;
if (transform) transform.hideFlags = HideFlags.None;
}
public static void RemoveContainerFlags(Component component)
{
if (component) component.hideFlags = HideFlags.None;
}
}
}
| {
"pile_set_name": "Github"
} |
/* ###
* IP: GHIDRA
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ghidra.app.util.pdb.pdbapplicator;
import java.math.BigInteger;
import java.util.*;
import ghidra.app.util.SymbolPath;
import ghidra.app.util.SymbolPathParser;
import ghidra.app.util.bin.format.pdb.DefaultCompositeMember;
import ghidra.app.util.bin.format.pdb2.pdbreader.*;
import ghidra.app.util.bin.format.pdb2.pdbreader.type.*;
import ghidra.program.model.data.*;
import ghidra.util.Msg;
import ghidra.util.exception.AssertException;
import ghidra.util.exception.CancelledException;
/*
* Non java-doc:
* Some truths:
* For AbstractMsCompositeType: do not count on "count" to be zero when MsProperty is forward
* reference (we have seen example of count==0 and not forward reference, though the majority
* of the time the two go hand-in-hand. When these did not equate, it might have been when
* there was no need for a forward reference and possibly only one definition--this would
* require a closer look).
*/
/**
* Applier for {@link AbstractCompositeMsType} types.
*/
public class CompositeTypeApplier extends AbstractComplexTypeApplier {
// DO NOT DELETE. Might eliminate one path or might make an analyzer option.
private static boolean applyBaseClasses = true;
//private static boolean applyBaseClasses = false;
private CppCompositeType classType;
// private final static DataType NO_TYPE_DATATYPE =
// new TypedefDataType("<NoType>", Undefined1DataType.dataType);
//
private Map<Integer, String> componentComments;
private List<DefaultPdbUniversalMember> members;
/**
* Constructor for composite type applier, for transforming a composite into a
* Ghidra DataType.
* @param applicator {@link PdbApplicator} for which this class is working.
* @param msType {@link AbstractCompositeMsType} to process.
*/
public CompositeTypeApplier(PdbApplicator applicator, AbstractCompositeMsType msType) {
super(applicator, msType);
String fullPathName = msType.getName();
symbolPath = new SymbolPath(SymbolPathParser.parse(fullPathName));
}
CppCompositeType getClassType() {
if (definitionApplier != null) {
return ((CompositeTypeApplier) definitionApplier).getClassTypeInternal();
}
return classType;
}
CppCompositeType getClassTypeInternal() {
return classType;
}
List<DefaultPdbUniversalMember> getMembers() {
return members;
}
// Mapping of forwardReference/definition must be done prior to this call.
private void getOrCreateComposite() {
if (dataType != null) {
return;
}
AbstractComplexTypeApplier alternativeApplier = getAlternativeTypeApplier();
if (alternativeApplier != null) {
dataType = alternativeApplier.getDataTypeInternal();
classType = ((CompositeTypeApplier) alternativeApplier).getClassTypeInternal();
}
if (dataType != null) {
return;
}
dataType = createEmptyComposite((AbstractCompositeMsType) msType);
String mangledName = ((AbstractCompositeMsType) msType).getMangledName();
classType = new CppCompositeType((Composite) dataType, mangledName);
classType.setName(getName());
classType.setSize(PdbApplicator.bigIntegerToInt(applicator, getSize()));
if (msType instanceof AbstractClassMsType) {
classType.setClass();
}
else if (msType instanceof AbstractStructureMsType) {
classType.setStruct();
}
else if (msType instanceof AbstractUnionMsType) {
classType.setUnion();
}
classType.setFinal(isFinal());
}
@Override
void apply() throws PdbException, CancelledException {
getOrCreateComposite();
Composite composite = (Composite) dataType;
AbstractCompositeMsType type = (AbstractCompositeMsType) msType;
MsProperty property = type.getMsProperty();
if (property.isForwardReference() && definitionApplier != null) {
return;
}
if (!(composite instanceof CompositeDataTypeImpl)) {
return; // A resolved version exists (multiple definitions).
}
applyOrDeferForDependencies();
}
@Override
void resolve() {
if (!isForwardReference()) {
super.resolve();
}
}
private void applyOrDeferForDependencies() throws PdbException, CancelledException {
AbstractCompositeMsType type = (AbstractCompositeMsType) msType;
MsProperty property = type.getMsProperty();
if (property.isForwardReference() && definitionApplier != null) {
return;
}
// Add self
applicator.addApplierDependency(this);
// Add any dependees: base classes and members
FieldListTypeApplier fieldListApplier = FieldListTypeApplier.getFieldListApplierSpecial(
applicator, type.getFieldDescriptorListRecordNumber());
// Currently do not need this dependency, as we currently do not need any contents
// of the base class for filling in this class
for (MsTypeApplier baseApplierIterated : fieldListApplier.getBaseClassList()) {
if (baseApplierIterated instanceof BaseClassTypeApplier) {
BaseClassTypeApplier baseTypeApplier = (BaseClassTypeApplier) baseApplierIterated;
MsTypeApplier applier =
applicator.getTypeApplier(baseTypeApplier.getBaseClassRecordNumber());
if (applier instanceof CompositeTypeApplier) {
CompositeTypeApplier dependencyApplier =
((CompositeTypeApplier) applier).getDependencyApplier();
applicator.addApplierDependency(this, dependencyApplier);
// CompositeTypeApplier defApplier =
// ((CompositeTypeApplier) applier).getDefinitionApplier();
// if (defApplier != null) {
// applicator.addApplierDependency(this, defApplier);
// }
// else {
// applicator.addApplierDependency(this, applier);
// }
setDeferred();
}
}
}
for (MsTypeApplier memberTypeApplierIterated : fieldListApplier.getMemberList()) {
applicator.checkCanceled();
if (memberTypeApplierIterated instanceof MemberTypeApplier) {
MemberTypeApplier memberTypeApplier = (MemberTypeApplier) memberTypeApplierIterated;
MsTypeApplier fieldApplier = memberTypeApplier.getFieldTypeApplier();
recurseAddDependency(fieldApplier);
}
// if (memberTypeApplierIterated instanceof NestedTypeApplier) {
// recurseAddDependency(memberTypeApplierIterated);
// }
else if (memberTypeApplierIterated instanceof VirtualFunctionTablePointerTypeApplier) {
applicator.addApplierDependency(this, memberTypeApplierIterated);
}
}
if (!isDeferred()) {
applyInternal();
}
}
private void recurseAddDependency(MsTypeApplier dependee)
throws CancelledException, PdbException {
// TODO: evaluate this and make changes... this work might be being taken care of in
// ModifierTypeApplier
if (dependee instanceof ModifierTypeApplier) {
ModifierTypeApplier modifierApplier = (ModifierTypeApplier) dependee;
recurseAddDependency(modifierApplier.getModifiedTypeApplier());
}
else if (dependee instanceof CompositeTypeApplier) {
CompositeTypeApplier defApplier =
((CompositeTypeApplier) dependee).getDefinitionApplier(CompositeTypeApplier.class);
if (defApplier != null) {
applicator.addApplierDependency(this, defApplier);
}
else {
applicator.addApplierDependency(this, dependee);
}
setDeferred();
}
// TODO: evaluate this and make changes... this work might be being taken care of in
// ArrayTypeApplier
else if (dependee instanceof ArrayTypeApplier) {
applicator.addApplierDependency(this, dependee);
setDeferred();
}
// else if (dependee instanceof NestedTypeApplier) {
// NestedTypeApplier nestedTypeApplier = (NestedTypeApplier) dependee;
// AbstractMsTypeApplier nestedDefinitionApplier =
// nestedTypeApplier.getNestedTypeDefinitionApplier();
// // Need to make sure that "this" class id dependent on all elements composing the
// // nested definition, but we need to create the nested definition during the
// // creation of this class. (NestedTypeApplier and NestedTypeMsType do not really
// // have their own RecordNumber).
// applicator.addApplierDependency(this, nestedDefinitionApplier);
// setDeferred();
// }
else if (dependee instanceof BitfieldTypeApplier) {
RecordNumber recNum =
((AbstractBitfieldMsType) ((BitfieldTypeApplier) dependee).getMsType()).getElementRecordNumber();
MsTypeApplier underlyingApplier = applicator.getTypeApplier(recNum);
if (underlyingApplier instanceof EnumTypeApplier) {
applicator.addApplierDependency(this, underlyingApplier);
setDeferred();
}
}
//We are assuming that bitfields on typedefs will not be defined.
}
private void applyInternal() throws CancelledException, PdbException {
if (isApplied()) {
return;
}
Composite composite = (Composite) dataType;
AbstractCompositeMsType type = (AbstractCompositeMsType) msType;
boolean applyCpp = applyBaseClasses;
if (type instanceof AbstractUnionMsType) {
applyCpp = false;
if (hasBaseClasses()) {
pdbLogAndInfoMessage(this,
"Unexpected base classes for union type: " + type.getName());
}
}
if (applyCpp) {
applyCpp(composite, type);
}
else {
applyBasic(composite, type);
}
setApplied();
}
//==============================================================================================
private void applyBasic(Composite composite, AbstractCompositeMsType type)
throws CancelledException, PdbException {
//boolean isClass = (type instanceof AbstractClassMsType || actsLikeClass(applicator, type));
boolean isClass = (type instanceof AbstractClassMsType);
int size = getSizeInt();
// Fill in composite definition details.
FieldListTypeApplier fieldListApplier = FieldListTypeApplier.getFieldListApplierSpecial(
applicator, type.getFieldDescriptorListRecordNumber());
clearComponents(composite);
members = new ArrayList<>();
componentComments = new HashMap<>();
addMembers(composite, fieldListApplier);
if (!DefaultCompositeMember.applyDataTypeMembers(composite, isClass, size, members,
msg -> reconstructionWarn(msg), applicator.getCancelOnlyWrappingMonitor())) {
clearComponents(composite);
}
setComponentComments(composite);
}
private void setComponentComments(Composite composite) {
if (composite instanceof Structure) {
Structure structure = (Structure) composite;
for (Map.Entry<Integer, String> entry : componentComments.entrySet()) {
DataTypeComponent component = structure.getComponentAt(entry.getKey());
if (component == null) {
pdbLogAndInfoMessage(this, "Could not set comment for 'missing' componenent " +
entry.getKey() + " for: " + structure.getName());
return;
}
component.setComment(entry.getValue());
}
}
}
//==============================================================================================
private void applyCpp(Composite composite, AbstractCompositeMsType type)
throws PdbException, CancelledException {
// Fill in composite definition details.
FieldListTypeApplier fieldListApplier = FieldListTypeApplier.getFieldListApplierSpecial(
applicator, type.getFieldDescriptorListRecordNumber());
clearComponents(composite);
members = new ArrayList<>(); // TODO: temporary for old "basic" mechanism
componentComments = new HashMap<>(); // TODO: temporary for old "basic" mechanism
addClassTypeBaseClasses(composite, fieldListApplier);
addMembers(composite, fieldListApplier);
if (!classType.validate()) {
// TODO: Investigate. We should do this check for some classes somewhere. Should
// we do it here. Set breakpoint here to investigate.
}
classType.createLayout(applicator.getPdbApplicatorOptions().getClassLayout(),
applicator.getVbtManager(), applicator.getCancelOnlyWrappingMonitor());
}
//==============================================================================================
private void reconstructionWarn(String msg) {
//TODO: if statement/contents temporary
if (msg.contains("failed to align") && hasHiddenComponents()) {
msg = msg.replaceFirst("PDB", "PDB CLASS");
}
Msg.warn(this, msg);
}
//==============================================================================================
@Override
void deferredApply() throws PdbException, CancelledException {
if (isDeferred()) {
applyInternal();
}
}
//==============================================================================================
//==============================================================================================
@Override
CompositeTypeApplier getDependencyApplier() {
if (definitionApplier != null && definitionApplier instanceof CompositeTypeApplier) {
return (CompositeTypeApplier) definitionApplier;
}
return this;
}
String getName() {
return getMsType().getName();
}
@Override
DataType getDataType() {
if (resolved) {
return resolvedDataType;
}
getOrCreateComposite();
return dataType;
}
@Override
DataType getCycleBreakType() {
if (isForwardReference() && definitionApplier != null && definitionApplier.isApplied()) {
return definitionApplier.getDataType();
}
return dataType;
}
boolean hasUniqueName() {
return ((AbstractCompositeMsType) msType).getMsProperty().hasUniqueName();
}
@Override
BigInteger getSize() {
return ((AbstractCompositeMsType) getDependencyApplier().getMsType()).getSize();
}
// TODO:
// Taken from PdbUtil without change. Would have had to change access on class PdbUtil and
// this ensureSize method to public to make it accessible. Can revert to using PdbUtil
// once we move this new module from Contrib to Features/PDB.
final static void clearComponents(Composite composite) {
if (composite instanceof Structure) {
((Structure) composite).deleteAll();
}
else {
while (composite.getNumComponents() > 0) {
composite.delete(0);
}
}
}
private Composite createEmptyComposite(AbstractCompositeMsType type) {
SymbolPath fixedPath = getFixedSymbolPath();
CategoryPath categoryPath = applicator.getCategory(fixedPath.getParent());
Composite composite;
if (type instanceof AbstractClassMsType) {
applicator.predefineClass(fixedPath);
composite = new StructureDataType(categoryPath, fixedPath.getName(), 0,
applicator.getDataTypeManager());
}
else if (type instanceof AbstractStructureMsType) {
composite = new StructureDataType(categoryPath, fixedPath.getName(), 0,
applicator.getDataTypeManager());
}
else if (type instanceof AbstractUnionMsType) {
composite = new UnionDataType(categoryPath, fixedPath.getName(),
applicator.getDataTypeManager());
}
else { // InterfaceMsType
String message = "Unsupported datatype (" + type.getClass().getSimpleName() + "): " +
fixedPath.getPath();
applicator.appendLogMsg(message);
return null;
}
return composite;
}
private boolean hasBaseClasses() {
AbstractCompositeMsType defType;
if (definitionApplier == null) {
if (isForwardReference()) {
return false;
}
defType = (AbstractCompositeMsType) msType;
}
else {
defType = (AbstractCompositeMsType) definitionApplier.getMsType();
}
MsTypeApplier applier =
applicator.getTypeApplier(defType.getFieldDescriptorListRecordNumber());
if (!(applier instanceof FieldListTypeApplier)) {
return false;
}
FieldListTypeApplier fieldListApplier = (FieldListTypeApplier) applier;
AbstractFieldListMsType fieldListType =
((AbstractFieldListMsType) fieldListApplier.getMsType());
if (fieldListType.getBaseClassList().size() != 0) {
return true;
}
return (fieldListType.getBaseClassList().size() != 0);
}
private boolean hasHiddenComponents() {
AbstractCompositeMsType defType;
if (definitionApplier == null) {
if (isForwardReference()) {
return false;
}
defType = (AbstractCompositeMsType) msType;
}
else {
defType = (AbstractCompositeMsType) definitionApplier.getMsType();
}
// Note: if a "class" only has structure fields--does not have member functions, base
// class, virtual inheritance, etc., then it acts like a structure, meaning that there
// should be no extra fields for pvft, pvbt, base and virtual class components.
// So... it might not be good to return "true" for just checking if the type is an
// instanceof AbstractClassMsType.
MsTypeApplier applier =
applicator.getTypeApplier(defType.getFieldDescriptorListRecordNumber());
if (!(applier instanceof FieldListTypeApplier)) {
return false;
}
FieldListTypeApplier fieldListApplier = (FieldListTypeApplier) applier;
AbstractFieldListMsType fieldListType =
((AbstractFieldListMsType) fieldListApplier.getMsType());
return (fieldListType.getMethodList().size() != 0 ||
fieldListType.getBaseClassList().size() != 0);
}
private void addClassTypeBaseClasses(Composite composite, FieldListTypeApplier fieldListApplier)
throws PdbException {
AbstractCompositeMsType type = (AbstractCompositeMsType) msType;
for (MsTypeApplier baseApplierIterated : fieldListApplier.getBaseClassList()) {
if (!(baseApplierIterated instanceof BaseClassTypeApplier)) {
applicator.appendLogMsg(baseApplierIterated.getClass().getSimpleName() +
" seen where BaseClassTypeApplier expected for " + type.getName());
continue;
}
BaseClassTypeApplier baseTypeApplier = (BaseClassTypeApplier) baseApplierIterated;
MsTypeApplier baseClassTypeApplier =
applicator.getTypeApplier(baseTypeApplier.getBaseClassRecordNumber());
if (!(baseClassTypeApplier instanceof CompositeTypeApplier)) {
applicator.appendLogMsg(baseApplierIterated.getClass().getSimpleName() +
" seen where CompositeTypeApplier expected for " + type.getName());
continue;
}
AbstractMsType baseClassMsType = baseTypeApplier.getMsType();
if (baseClassMsType instanceof AbstractBaseClassMsType) {
applyDirectBaseClass((AbstractBaseClassMsType) baseClassMsType);
}
else if (baseClassMsType instanceof AbstractVirtualBaseClassMsType) {
applyDirectVirtualBaseClass((AbstractVirtualBaseClassMsType) baseClassMsType);
}
else if (baseClassMsType instanceof AbstractIndirectVirtualBaseClassMsType) {
applyIndirectVirtualBaseClass(
(AbstractIndirectVirtualBaseClassMsType) baseClassMsType);
}
else {
throw new AssertException(
"Unknown base class type: " + baseClassMsType.getClass().getSimpleName());
}
}
}
private void applyDirectBaseClass(AbstractBaseClassMsType base) throws PdbException {
CppCompositeType underlyingClassType =
getUnderlyingClassType(base.getBaseClassRecordNumber());
if (underlyingClassType == null) {
return;
}
ClassFieldMsAttributes atts = base.getAttributes();
int offset = PdbApplicator.bigIntegerToInt(applicator, base.getOffset());
classType.addDirectBaseClass(underlyingClassType, convertAttributes(atts), offset);
}
private void applyDirectVirtualBaseClass(AbstractVirtualBaseClassMsType base)
throws PdbException {
CppCompositeType underlyingCt = getUnderlyingClassType(base.getBaseClassRecordNumber());
if (underlyingCt == null) {
return;
}
DataType vbtptr =
getVirtualBaseTablePointerDataType(base.getVirtualBasePointerRecordNumber());
ClassFieldMsAttributes atts = base.getAttributes();
int basePointerOffset =
PdbApplicator.bigIntegerToInt(applicator, base.getBasePointerOffset());
int offsetFromVbt = PdbApplicator.bigIntegerToInt(applicator, base.getBaseOffsetFromVbt());
classType.addDirectVirtualBaseClass(underlyingCt, convertAttributes(atts),
basePointerOffset, vbtptr, offsetFromVbt);
}
private void applyIndirectVirtualBaseClass(AbstractIndirectVirtualBaseClassMsType base)
throws PdbException {
CppCompositeType underlyingCt = getUnderlyingClassType(base.getBaseClassRecordNumber());
if (underlyingCt == null) {
return;
}
DataType vbtptr =
getVirtualBaseTablePointerDataType(base.getVirtualBasePointerRecordNumber());
ClassFieldMsAttributes atts = base.getAttributes();
int basePointerOffset =
PdbApplicator.bigIntegerToInt(applicator, base.getBasePointerOffset());
int offsetFromVbt = PdbApplicator.bigIntegerToInt(applicator, base.getBaseOffsetFromVbt());
classType.addIndirectVirtualBaseClass(underlyingCt, convertAttributes(atts),
basePointerOffset, vbtptr, offsetFromVbt);
}
private CppCompositeType getUnderlyingClassType(RecordNumber recordNumber) {
MsTypeApplier baseUnderlyingApplier = applicator.getTypeApplier(recordNumber);
if (!(baseUnderlyingApplier instanceof CompositeTypeApplier)) {
applicator.appendLogMsg(baseUnderlyingApplier.getClass().getSimpleName() +
" seen where CompositeTypeApplier expected for base class.");
return null;
}
CompositeTypeApplier baseApplier = (CompositeTypeApplier) baseUnderlyingApplier;
CppCompositeType underlyingClassType = baseApplier.getClassType();
if (underlyingClassType == null) {
applicator.appendLogMsg("Underlying base class type is null.");
}
return underlyingClassType;
}
private DataType getVirtualBaseTablePointerDataType(RecordNumber recordNumber) {
MsTypeApplier vbptrApplier = applicator.getTypeApplier(recordNumber);
if (vbptrApplier != null) {
if (vbptrApplier instanceof PointerTypeApplier) {
return vbptrApplier.getDataType();
}
}
applicator.appendLogMsg("Generating a generic Virtual Base Table Pointer.");
return new PointerDataType();
}
private static CppCompositeType.ClassFieldAttributes convertAttributes(
ClassFieldMsAttributes atts) {
CppCompositeType.Access myAccess;
switch (atts.getAccess()) {
case PUBLIC:
myAccess = CppCompositeType.Access.PUBLIC;
break;
case PROTECTED:
myAccess = CppCompositeType.Access.PROTECTED;
break;
case PRIVATE:
myAccess = CppCompositeType.Access.PRIVATE;
break;
default:
myAccess = CppCompositeType.Access.BLANK;
break;
}
CppCompositeType.Property myProperty;
switch (atts.getProperty()) {
case VIRTUAL:
myProperty = CppCompositeType.Property.VIRTUAL;
break;
case STATIC:
myProperty = CppCompositeType.Property.STATIC;
break;
case FRIEND:
myProperty = CppCompositeType.Property.FRIEND;
break;
default:
myProperty = CppCompositeType.Property.BLANK;
break;
}
return new CppCompositeType.ClassFieldAttributes(myAccess, myProperty);
}
private void addMembers(Composite composite, FieldListTypeApplier fieldListApplier) {
AbstractCompositeMsType type = (AbstractCompositeMsType) msType;
for (MsTypeApplier memberTypeApplierIterated : fieldListApplier.getMemberList()) {
boolean handled = true;
if (memberTypeApplierIterated instanceof MemberTypeApplier) {
MemberTypeApplier memberTypeApplier = (MemberTypeApplier) memberTypeApplierIterated;
String memberName = memberTypeApplier.getName();
int offset =
PdbApplicator.bigIntegerToInt(applicator, memberTypeApplier.getOffset());
ClassFieldMsAttributes memberAttributes = memberTypeApplier.getAttribute();
memberAttributes.getAccess(); // TODO: do something with this and other attributes
MsTypeApplier fieldApplier = memberTypeApplier.getFieldTypeApplier();
if (fieldApplier instanceof CompositeTypeApplier) {
CompositeTypeApplier defApplier =
((CompositeTypeApplier) fieldApplier).getDefinitionApplier(
CompositeTypeApplier.class);
if (defApplier != null) {
fieldApplier = defApplier;
}
}
DataType fieldDataType = fieldApplier.getDataType();
boolean isFlexibleArray;
if (fieldApplier instanceof ArrayTypeApplier) {
isFlexibleArray = ((ArrayTypeApplier) fieldApplier).isFlexibleArray();
}
else {
isFlexibleArray = false;
}
if (fieldDataType == null) {
if (fieldApplier instanceof PrimitiveTypeApplier &&
((PrimitiveTypeApplier) fieldApplier).isNoType()) {
DefaultPdbUniversalMember member = new DefaultPdbUniversalMember(applicator,
memberName, fieldApplier, offset);
members.add(member);
componentComments.put(offset, "NO_TYPE");
}
else {
applicator.appendLogMsg("PDB Warning: No conversion for " + memberName +
" " + fieldApplier.getMsType().getClass().getSimpleName() +
" in composite " + composite.getName());
}
}
else {
DefaultPdbUniversalMember member =
new DefaultPdbUniversalMember(applicator, memberName, fieldApplier, offset);
members.add(member);
classType.addMember(memberName, fieldDataType, isFlexibleArray,
convertAttributes(memberAttributes), offset);
}
}
else if (memberTypeApplierIterated instanceof EnumerateTypeApplier) {
EnumerateTypeApplier enumerateTypeApplier =
(EnumerateTypeApplier) memberTypeApplierIterated;
String fieldName = enumerateTypeApplier.getName();
Numeric numeric = enumerateTypeApplier.getNumeric();
// TODO: some work
pdbLogAndInfoMessage(this,
"Don't know how to apply EnumerateTypeApplier fieldName " + fieldName +
" and value " + numeric + " within " + msType.getName());
}
else if (memberTypeApplierIterated instanceof VirtualFunctionTablePointerTypeApplier) {
VirtualFunctionTablePointerTypeApplier vtPtrApplier =
(VirtualFunctionTablePointerTypeApplier) memberTypeApplierIterated;
String vftPtrMemberName = vtPtrApplier.getMemberName();
int offset = vtPtrApplier.getOffset();
DefaultPdbUniversalMember member = new DefaultPdbUniversalMember(applicator,
vftPtrMemberName, vtPtrApplier, offset);
members.add(member);
//classType.addMember(vftPtrMemberName, vtPtrApplier.getDataType(), false, offset);
classType.addVirtualFunctionTablePointer(vftPtrMemberName,
vtPtrApplier.getDataType(), offset);
}
else if (memberTypeApplierIterated instanceof NestedTypeApplier) {
// Need to make sure that "this" class id dependent on all elements composing the
// nested definition, but we need to create the nested definition during the
// creation of this class. (NestedTypeApplier and NestedTypeMsType do not really
// have their own RecordNumber).
// 20200114: think this is a nested typedef.
NestedTypeApplier nestedTypeApplier = (NestedTypeApplier) memberTypeApplierIterated;
String memberTypeName = nestedTypeApplier.getTypeName();
String memberName = nestedTypeApplier.getMemberName(); // use this
// TODO: we are assuming that the offset is zero (0) for the call. Need to dig
// more to confirm this. Is ever anything but just one nested type? The pdb.exe
// generates these all at offset 0.
// TODO: Nested types are currently an issue for
// DefaultCompositeMember.applyDataTypeMembers().
// Need to investigate what to do here. It could be just when the specific
// composite is a member of itself.
if (type.getName().equals(memberTypeName)) {
// We are skipping because we've had issues and do not know what is going on
// at the moment. (I think they were dependency issues... been a while.)
// See not above the "if" condition.
// pdbLogAndInfoMessage(this, "Skipping Composite Nested type member: " +
// memberName + " within " + type.getName());
// TODO: Investigate. What does it mean when the internally defined type
// conficts with the name of the outer type.
continue;
}
// TODO: believe the thing to do is to show that these are types that are
// defined within the namespace of this containing type. This might be
// the place to do it... that is if we don't identify them separately
// falling under the namespace of this composite.
// AbstractMsTypeApplier nestedDefinitionApplier =
// nestedTypeApplier.getNestedTypeDefinitionApplier().getDependencyApplier();
//
// DataType ndt = nestedDefinitionApplier.getDataType(); //use this
// int ndtl = ndt.getLength(); //use this
//
// AbstractMsType ndms = nestedTypeApplier.getMsType();
//
// BigInteger val = nestedTypeApplier.getSize();
// int offset = 0; // ???? TODO..,
// DataType nt = nestedTypeApplier.getDataType();
// ClassFieldMsAttributes a = nestedTypeApplier.getAttributes();
//
// // TODO: hoping this is right... 20200521... how/where do we get offset?
// Default2PdbMember member =
// new Default2PdbMember(applicator, memberName, nestedDefinitionApplier, offset);
// members.add(member);
}
else if (memberTypeApplierIterated instanceof NoTypeApplier) {
AbstractMsType msNoType = memberTypeApplierIterated.getMsType();
if (msNoType instanceof AbstractStaticMemberMsType) {
// TODO: Investigate anything that hits here (set break point), see if we
// see dot apply the information. If so, probably should create an applier
// for the contained MS type.
}
else {
handled = false;
}
}
else {
handled = false;
}
if (!handled) {
applicator.appendLogMsg(
memberTypeApplierIterated.getClass().getSimpleName() + " with contained " +
memberTypeApplierIterated.getMsType().getClass().getSimpleName() +
" unexpected for " + msType.getName());
}
}
}
// /**
// * <code>NoType</code> provides ability to hang NoType into a composite type by faking
// * it with a zero-length bitfield. This is a bit of a kludge
// * This will be transformed to a normal BitFieldDataType when cloned.
// */
// private class NoType extends PdbBitField {
// private NoType(PdbApplicator applicator) throws InvalidDataTypeException {
// super(new CharDataType(applicator.getDataTypeManager()), 0, 0);
// }
// }
//
}
| {
"pile_set_name": "Github"
} |
/* Cholesky Decomposition
*
* Copyright (C) 2000 Thomas Walter
*
* 03 May 2000: Modified for GSL by Brian Gough
* 29 Jul 2005: Additions by Gerard Jungman
* Copyright (C) 2000,2001, 2002, 2003, 2005, 2007 Brian Gough, Gerard Jungman
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License as published by the
* Free Software Foundation; either version 3, or (at your option) any
* later version.
*
* This source is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* for more details.
*/
/*
* Cholesky decomposition of a symmetrix positive definite matrix.
* This is useful to solve the matrix arising in
* periodic cubic splines
* approximating splines
*
* This algorithm does:
* A = L * L'
* with
* L := lower left triangle matrix
* L' := the transposed form of L.
*
*/
#include "gsl__config.h"
#include "gsl_math.h"
#include "gsl_errno.h"
#include "gsl_vector.h"
#include "gsl_matrix.h"
#include "gsl_blas.h"
#include "gsl_linalg.h"
static inline
double
quiet_sqrt (double x)
/* avoids runtime error, for checking matrix for positive definiteness */
{
return (x >= 0) ? sqrt(x) : GSL_NAN;
}
int
gsl_linalg_cholesky_decomp (gsl_matrix * A)
{
const size_t M = A->size1;
const size_t N = A->size2;
if (M != N)
{
GSL_ERROR("cholesky decomposition requires square matrix", GSL_ENOTSQR);
}
else
{
size_t i,j,k;
int status = 0;
/* Do the first 2 rows explicitly. It is simple, and faster. And
* one can return if the matrix has only 1 or 2 rows.
*/
double A_00 = gsl_matrix_get (A, 0, 0);
double L_00 = quiet_sqrt(A_00);
if (A_00 <= 0)
{
status = GSL_EDOM ;
}
gsl_matrix_set (A, 0, 0, L_00);
if (M > 1)
{
double A_10 = gsl_matrix_get (A, 1, 0);
double A_11 = gsl_matrix_get (A, 1, 1);
double L_10 = A_10 / L_00;
double diag = A_11 - L_10 * L_10;
double L_11 = quiet_sqrt(diag);
if (diag <= 0)
{
status = GSL_EDOM;
}
gsl_matrix_set (A, 1, 0, L_10);
gsl_matrix_set (A, 1, 1, L_11);
}
for (k = 2; k < M; k++)
{
double A_kk = gsl_matrix_get (A, k, k);
for (i = 0; i < k; i++)
{
double sum = 0;
double A_ki = gsl_matrix_get (A, k, i);
double A_ii = gsl_matrix_get (A, i, i);
gsl_vector_view ci = gsl_matrix_row (A, i);
gsl_vector_view ck = gsl_matrix_row (A, k);
if (i > 0) {
gsl_vector_view di = gsl_vector_subvector(&ci.vector, 0, i);
gsl_vector_view dk = gsl_vector_subvector(&ck.vector, 0, i);
gsl_blas_ddot (&di.vector, &dk.vector, &sum);
}
A_ki = (A_ki - sum) / A_ii;
gsl_matrix_set (A, k, i, A_ki);
}
{
gsl_vector_view ck = gsl_matrix_row (A, k);
gsl_vector_view dk = gsl_vector_subvector (&ck.vector, 0, k);
double sum = gsl_blas_dnrm2 (&dk.vector);
double diag = A_kk - sum * sum;
double L_kk = quiet_sqrt(diag);
if (diag <= 0)
{
status = GSL_EDOM;
}
gsl_matrix_set (A, k, k, L_kk);
}
}
/* Now copy the transposed lower triangle to the upper triangle,
* the diagonal is common.
*/
for (i = 1; i < M; i++)
{
for (j = 0; j < i; j++)
{
double A_ij = gsl_matrix_get (A, i, j);
gsl_matrix_set (A, j, i, A_ij);
}
}
if (status == GSL_EDOM)
{
GSL_ERROR ("matrix must be positive definite", GSL_EDOM);
}
return GSL_SUCCESS;
}
}
int
gsl_linalg_cholesky_solve (const gsl_matrix * LLT,
const gsl_vector * b,
gsl_vector * x)
{
if (LLT->size1 != LLT->size2)
{
GSL_ERROR ("cholesky matrix must be square", GSL_ENOTSQR);
}
else if (LLT->size1 != b->size)
{
GSL_ERROR ("matrix size must match b size", GSL_EBADLEN);
}
else if (LLT->size2 != x->size)
{
GSL_ERROR ("matrix size must match solution size", GSL_EBADLEN);
}
else
{
/* Copy x <- b */
gsl_vector_memcpy (x, b);
/* Solve for c using forward-substitution, L c = b */
gsl_blas_dtrsv (CblasLower, CblasNoTrans, CblasNonUnit, LLT, x);
/* Perform back-substitution, U x = c */
gsl_blas_dtrsv (CblasUpper, CblasNoTrans, CblasNonUnit, LLT, x);
return GSL_SUCCESS;
}
}
int
gsl_linalg_cholesky_svx (const gsl_matrix * LLT,
gsl_vector * x)
{
if (LLT->size1 != LLT->size2)
{
GSL_ERROR ("cholesky matrix must be square", GSL_ENOTSQR);
}
else if (LLT->size2 != x->size)
{
GSL_ERROR ("matrix size must match solution size", GSL_EBADLEN);
}
else
{
/* Solve for c using forward-substitution, L c = b */
gsl_blas_dtrsv (CblasLower, CblasNoTrans, CblasNonUnit, LLT, x);
/* Perform back-substitution, U x = c */
gsl_blas_dtrsv (CblasUpper, CblasNoTrans, CblasNonUnit, LLT, x);
return GSL_SUCCESS;
}
}
int
gsl_linalg_cholesky_decomp_unit(gsl_matrix * A, gsl_vector * D)
{
const size_t N = A->size1;
size_t i, j;
/* initial Cholesky */
int stat_chol = gsl_linalg_cholesky_decomp(A);
if(stat_chol == GSL_SUCCESS)
{
/* calculate D from diagonal part of initial Cholesky */
for(i = 0; i < N; ++i)
{
const double C_ii = gsl_matrix_get(A, i, i);
gsl_vector_set(D, i, C_ii*C_ii);
}
/* multiply initial Cholesky by 1/sqrt(D) on the right */
for(i = 0; i < N; ++i)
{
for(j = 0; j < N; ++j)
{
gsl_matrix_set(A, i, j, gsl_matrix_get(A, i, j) / sqrt(gsl_vector_get(D, j)));
}
}
/* Because the initial Cholesky contained both L and transpose(L),
the result of the multiplication is not symmetric anymore;
but the lower triangle _is_ correct. Therefore we reflect
it to the upper triangle and declare victory.
*/
for(i = 0; i < N; ++i)
for(j = i + 1; j < N; ++j)
gsl_matrix_set(A, i, j, gsl_matrix_get(A, j, i));
}
return stat_chol;
}
| {
"pile_set_name": "Github"
} |
{
"randomStatetest156" : {
"_info" : {
"comment" : "",
"filling-rpc-server" : "Geth-1.9.6-unstable-63b18027-20190920",
"filling-tool-version" : "retesteth-0.0.1+commit.0ae18aef.Linux.g++",
"lllcversion" : "Version: 0.5.12-develop.2019.9.13+commit.2d601a4f.Linux.g++",
"source" : "src/GeneralStateTestsFiller/stRandom/randomStatetest156Filler.json",
"sourceHash" : "14a77efbdd3c1333204a8d96d7b4eeaa2da4140a273eea1a94fcbb7a785a6538"
},
"env" : {
"currentCoinbase" : "0x945304eb96065b2a98b57a48a06ae28d285a71b5",
"currentDifficulty" : "0x020000",
"currentGasLimit" : "0x7fffffffffffffff",
"currentNumber" : "0x01",
"currentTimestamp" : "0x03e8",
"previousHash" : "0x5e20a0453cecd065ea59c37ac63e079ee08998b6045136a8ce6635c7912ec0b6"
},
"post" : {
"Istanbul" : [
{
"indexes" : {
"data" : 0,
"gas" : 0,
"value" : 0
},
"hash" : "0x6ef23709b984b9020918b7b7c58ba67db573f2aad7fdff2f52913af9c9d9a1b7",
"logs" : "0x1dcc4de8dec75d7aab85b567b6ccd41ad312451b948a7413f0a142fd40d49347"
}
]
},
"pre" : {
"0x095e7baea6a6c7c4c2dfeb977efac326af552d87" : {
"balance" : "0x00",
"code" : "0x7f00000000000000000000000000000000000000000000000000000000000000007f000000000000000000000000ffffffffffffffffffffffffffffffffffffffff7f000000000000000000000000ffffffffffffffffffffffffffffffffffffffff7f000000000000000000000000000000000000000000000000000000000000c3507f00000000000000000000000100000000000000000000000000000000000000007f000000000000000000000000000000000000000000000000000000000000c3506f813982583141966b389c159aa48b3a8860005155",
"nonce" : "0x00",
"storage" : {
}
},
"0x945304eb96065b2a98b57a48a06ae28d285a71b5" : {
"balance" : "0x2e",
"code" : "0x6000355415600957005b60203560003555",
"nonce" : "0x00",
"storage" : {
}
},
"0xa94f5374fce5edbc8e2a8697c15331677e6ebf0b" : {
"balance" : "0x0de0b6b3a7640000",
"code" : "0x",
"nonce" : "0x00",
"storage" : {
}
}
},
"transaction" : {
"data" : [
"0x7f00000000000000000000000000000000000000000000000000000000000000007f000000000000000000000000ffffffffffffffffffffffffffffffffffffffff7f000000000000000000000000ffffffffffffffffffffffffffffffffffffffff7f000000000000000000000000000000000000000000000000000000000000c3507f00000000000000000000000100000000000000000000000000000000000000007f000000000000000000000000000000000000000000000000000000000000c3506f813982583141966b389c159aa48b3a88"
],
"gasLimit" : [
"0x244eb85c"
],
"gasPrice" : "0x01",
"nonce" : "0x00",
"secretKey" : "0x45a915e4d060149eb4365960e6a7a45f334393093061116b197e3240065ff2d8",
"to" : "0x095e7baea6a6c7c4c2dfeb977efac326af552d87",
"value" : [
"0x7ffe6411"
]
}
}
} | {
"pile_set_name": "Github"
} |
/*
* Copyright 2020 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* External dependencies
*/
import { select } from '@storybook/addon-knobs';
import { action } from '@storybook/addon-actions';
/**
* Internal dependencies
*/
import { VIEW_STYLE } from '../../../constants';
import ViewStyleBar from '..';
export default {
title: 'Dashboard/Components/ViewStyleBar',
component: ViewStyleBar,
};
export const _default = () => {
return (
<ViewStyleBar
layoutStyle={select('layoutStyle', VIEW_STYLE, VIEW_STYLE.LIST)}
onPress={action('on press clicked')}
/>
);
};
| {
"pile_set_name": "Github"
} |
var streamUrl = "https://streamer.cryptocompare.com/";
var fsym = "BTC";
var tsym = "USD";
var currentSubs;
var currentSubsText = "";
var dataUrl = "https://min-api.cryptocompare.com/data/subs?fsym=" + fsym + "&tsyms=" + tsym;
var socket = io(streamUrl);
$.getJSON(dataUrl, function(data) {
currentSubs = data['USD']['TRADES'];
console.log(currentSubs);
for (var i = 0; i < currentSubs.length; i++) {
currentSubsText += currentSubs[i] + ", ";
}
$('#sub-exchanges').text(currentSubsText);
socket.emit('SubAdd', { subs: currentSubs });
});
socket.on('m', function(currentData) {
var tradeField = currentData.substr(0, currentData.indexOf("~"));
if (tradeField == CCC.STATIC.TYPE.TRADE) {
transformData(currentData);
}
});
var transformData = function(data) {
var coinfsym = CCC.STATIC.CURRENCY.getSymbol(fsym);
var cointsym = CCC.STATIC.CURRENCY.getSymbol(tsym)
var incomingTrade = CCC.TRADE.unpack(data);
console.log(incomingTrade);
var newTrade = {
Market: incomingTrade['M'],
Type: incomingTrade['T'],
ID: incomingTrade['ID'],
Price: CCC.convertValueToDisplay(cointsym, incomingTrade['P']),
Quantity: CCC.convertValueToDisplay(coinfsym, incomingTrade['Q']),
Total: CCC.convertValueToDisplay(cointsym, incomingTrade['TOTAL'])
};
if (incomingTrade['F'] & 1) {
newTrade['Type'] = "SELL";
}
else if (incomingTrade['F'] & 2) {
newTrade['Type'] = "BUY";
}
else {
newTrade['Type'] = "UNKNOWN";
}
displayData(newTrade);
};
var displayData = function(dataUnpacked) {
var maxTableSize = 30;
var length = $('table tr').length;
$('#trades').after(
"<tr class=" + dataUnpacked.Type + "><th>" + dataUnpacked.Market + "</th><th>" + dataUnpacked.Type + "</th><th>" + dataUnpacked.ID + "</th><th>" + dataUnpacked.Price + "</th><th>" + dataUnpacked.Quantity + "</th><th>" + dataUnpacked.Total + "</th></tr>"
);
if (length >= (maxTableSize)) {
$('table tr:last').remove();
}
};
$('#unsubscribe').click(function() {
console.log('Unsubscribing to streamers');
$('#subscribe').removeClass('subon');
$(this).addClass('subon');
$('#stream-text').text('Stream stopped');
socket.emit('SubRemove', { subs: currentSubs });
$('#sub-exchanges').text("");
});
$('#subscribe').click(function() {
console.log('Subscribing to streamers')
$('#unsubscribe').removeClass('subon');
$(this).addClass('subon');
$('#stream-text').text("Streaming...");
socket.emit('SubAdd', { subs: currentSubs });
$('#sub-exchanges').text(currentSubsText);
}); | {
"pile_set_name": "Github"
} |
using System;
using System.Windows.Input;
namespace Samples.ViewModels
{
public class CommandViewModel
{
public string Text { get; set; }
public ICommand Command { get; set; }
}
}
| {
"pile_set_name": "Github"
} |
Zammad::Application.routes.draw do
# shorter version
match '/ical', to: 'calendar_subscriptions#all', via: :get
match '/ical/:object', to: 'calendar_subscriptions#object', via: :get
match '/ical/:object/:method', to: 'calendar_subscriptions#object', via: :get
# wording version
match '/calendar_subscriptions', to: 'calendar_subscriptions#all', via: :get
match '/calendar_subscriptions/:object', to: 'calendar_subscriptions#object', via: :get
match '/calendar_subscriptions/:object/:method', to: 'calendar_subscriptions#object', via: :get
end
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>BuildMachineOSBuild</key>
<string>18B57c</string>
<key>CFBundleDevelopmentRegion</key>
<string>English</string>
<key>CFBundleExecutable</key>
<string>ACPIBatteryManager</string>
<key>CFBundleGetInfoString</key>
<string>1.90.1, Copyright 2011 Apple Inc., RehabMan 2012</string>
<key>CFBundleIdentifier</key>
<string>org.rehabman.driver.AppleSmartBatteryManager</string>
<key>CFBundleInfoDictionaryVersion</key>
<string>6.0</string>
<key>CFBundleName</key>
<string>ACPIBatteryManager</string>
<key>CFBundlePackageType</key>
<string>KEXT</string>
<key>CFBundleShortVersionString</key>
<string>1.90.1-tluck</string>
<key>CFBundleSignature</key>
<string>????</string>
<key>CFBundleSupportedPlatforms</key>
<array>
<string>MacOSX</string>
</array>
<key>CFBundleVersion</key>
<string>1.90.1</string>
<key>DTCompiler</key>
<string>com.apple.compilers.llvm.clang.1_0</string>
<key>DTPlatformBuild</key>
<string>10A255</string>
<key>DTPlatformVersion</key>
<string>GM</string>
<key>DTSDKBuild</key>
<string>18A384</string>
<key>DTSDKName</key>
<string>macosx10.14</string>
<key>DTXcode</key>
<string>1000</string>
<key>DTXcodeBuild</key>
<string>10A255</string>
<key>IOKitPersonalities</key>
<dict>
<key>ACPI AC Adapter</key>
<dict>
<key>CFBundleIdentifier</key>
<string>org.rehabman.driver.AppleSmartBatteryManager</string>
<key>IOClass</key>
<string>ACPIACAdapter</string>
<key>IONameMatch</key>
<string>ACPI0003</string>
<key>IOProbeScore</key>
<integer>1000</integer>
<key>IOProviderClass</key>
<string>IOACPIPlatformDevice</string>
</dict>
<key>ACPI Battery Manager</key>
<dict>
<key>CFBundleIdentifier</key>
<string>org.rehabman.driver.AppleSmartBatteryManager</string>
<key>Configuration</key>
<dict>
<key>Correct16bitSignedCurrentRate</key>
<true/>
<key>CorrectCorruptCapacities</key>
<true/>
<key>CurrentDischargeRateMax</key>
<integer>20000</integer>
<key>EstimateCycleCountDivisor</key>
<integer>6</integer>
<key>FirstPollDelay</key>
<integer>2000</integer>
<key>StartupDelay</key>
<integer>8000</integer>
<key>UseDesignVoltageForCurrentCapacity</key>
<false/>
<key>UseDesignVoltageForDesignCapacity</key>
<false/>
<key>UseDesignVoltageForMaxCapacity</key>
<false/>
<key>UseExtendedBatteryInformationMethod</key>
<false/>
<key>UseExtraBatteryInformationMethod</key>
<false/>
</dict>
<key>IOClass</key>
<string>AppleSmartBatteryManager</string>
<key>IONameMatch</key>
<string>PNP0C0A</string>
<key>IOProbeScore</key>
<integer>1000</integer>
<key>IOProviderClass</key>
<string>IOACPIPlatformDevice</string>
</dict>
</dict>
<key>NSHumanReadableCopyright</key>
<string>Copyright © 2011 Apple Inc. All rights reserved, RehabMan 2012</string>
<key>OSBundleLibraries</key>
<dict>
<key>com.apple.iokit.IOACPIFamily</key>
<string>1.0d1</string>
<key>com.apple.kpi.iokit</key>
<string>9.0</string>
<key>com.apple.kpi.libkern</key>
<string>9.0</string>
</dict>
<key>OSBundleRequired</key>
<string>Root</string>
<key>Source Code</key>
<string>https://github.com/RehabMan/OS-X-ACPI-Battery-Driver</string>
</dict>
</plist>
| {
"pile_set_name": "Github"
} |
// Copyright Aleksey Gurtovoy 2000-2004
//
// Distributed under the Boost Software License, Version 1.0.
// (See accompanying file LICENSE_1_0.txt or copy at
// http://www.boost.org/LICENSE_1_0.txt)
//
// Preprocessed version of "boost/mpl/set_c.hpp" header
// -- DO NOT modify by hand!
namespace boost { namespace mpl {
template<
typename T, long C0 = LONG_MAX, long C1 = LONG_MAX, long C2 = LONG_MAX
, long C3 = LONG_MAX, long C4 = LONG_MAX, long C5 = LONG_MAX
, long C6 = LONG_MAX, long C7 = LONG_MAX, long C8 = LONG_MAX
, long C9 = LONG_MAX, long C10 = LONG_MAX, long C11 = LONG_MAX
, long C12 = LONG_MAX, long C13 = LONG_MAX, long C14 = LONG_MAX
, long C15 = LONG_MAX, long C16 = LONG_MAX, long C17 = LONG_MAX
, long C18 = LONG_MAX, long C19 = LONG_MAX
>
struct set_c;
template<
typename T
>
struct set_c<
T, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
>
: set0_c<T>
{
typedef typename set0_c<T>::type type;
};
template<
typename T, long C0
>
struct set_c<
T, C0, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
>
: set1_c< T,C0 >
{
typedef typename set1_c< T,C0 >::type type;
};
template<
typename T, long C0, long C1
>
struct set_c<
T, C0, C1, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
>
: set2_c< T,C0,C1 >
{
typedef typename set2_c< T,C0,C1 >::type type;
};
template<
typename T, long C0, long C1, long C2
>
struct set_c<
T, C0, C1, C2, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
>
: set3_c< T,C0,C1,C2 >
{
typedef typename set3_c< T,C0,C1,C2 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3
>
struct set_c<
T, C0, C1, C2, C3, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
>
: set4_c< T,C0,C1,C2,C3 >
{
typedef typename set4_c< T,C0,C1,C2,C3 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4
>
struct set_c<
T, C0, C1, C2, C3, C4, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
>
: set5_c< T,C0,C1,C2,C3,C4 >
{
typedef typename set5_c< T,C0,C1,C2,C3,C4 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX
>
: set6_c< T,C0,C1,C2,C3,C4,C5 >
{
typedef typename set6_c< T,C0,C1,C2,C3,C4,C5 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX
>
: set7_c< T,C0,C1,C2,C3,C4,C5,C6 >
{
typedef typename set7_c< T,C0,C1,C2,C3,C4,C5,C6 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX
>
: set8_c< T,C0,C1,C2,C3,C4,C5,C6,C7 >
{
typedef typename set8_c< T,C0,C1,C2,C3,C4,C5,C6,C7 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7, long C8
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX
>
: set9_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8 >
{
typedef typename set9_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7, long C8, long C9
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
, LONG_MAX
>
: set10_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9 >
{
typedef typename set10_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7, long C8, long C9, long C10
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, LONG_MAX, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
>
: set11_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10 >
{
typedef typename set11_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7, long C8, long C9, long C10, long C11
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
>
: set12_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10,C11 >
{
typedef typename set12_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10,C11 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7, long C8, long C9, long C10, long C11, long C12
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, LONG_MAX
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
>
: set13_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10,C11,C12 >
{
typedef typename set13_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10,C11,C12 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7, long C8, long C9, long C10, long C11, long C12
, long C13
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
>
: set14_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13
>
{
typedef typename set14_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10,C11,C12,C13 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7, long C8, long C9, long C10, long C11, long C12
, long C13, long C14
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13, C14
, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
>
: set15_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13, C14
>
{
typedef typename set15_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10,C11,C12,C13,C14 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7, long C8, long C9, long C10, long C11, long C12
, long C13, long C14, long C15
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13, C14
, C15, LONG_MAX, LONG_MAX, LONG_MAX, LONG_MAX
>
: set16_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13, C14
, C15
>
{
typedef typename set16_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10,C11,C12,C13,C14,C15 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7, long C8, long C9, long C10, long C11, long C12
, long C13, long C14, long C15, long C16
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13, C14
, C15, C16, LONG_MAX, LONG_MAX, LONG_MAX
>
: set17_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13, C14
, C15, C16
>
{
typedef typename set17_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10,C11,C12,C13,C14,C15,C16 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7, long C8, long C9, long C10, long C11, long C12
, long C13, long C14, long C15, long C16, long C17
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13, C14
, C15, C16, C17, LONG_MAX, LONG_MAX
>
: set18_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13, C14
, C15, C16, C17
>
{
typedef typename set18_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10,C11,C12,C13,C14,C15,C16,C17 >::type type;
};
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7, long C8, long C9, long C10, long C11, long C12
, long C13, long C14, long C15, long C16, long C17, long C18
>
struct set_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13, C14
, C15, C16, C17, C18, LONG_MAX
>
: set19_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13, C14
, C15, C16, C17, C18
>
{
typedef typename set19_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10,C11,C12,C13,C14,C15,C16,C17,C18 >::type type;
};
/// primary template (not a specialization!)
template<
typename T, long C0, long C1, long C2, long C3, long C4, long C5
, long C6, long C7, long C8, long C9, long C10, long C11, long C12
, long C13, long C14, long C15, long C16, long C17, long C18, long C19
>
struct set_c
: set20_c<
T, C0, C1, C2, C3, C4, C5, C6, C7, C8, C9, C10, C11, C12, C13, C14
, C15, C16, C17, C18, C19
>
{
typedef typename set20_c< T,C0,C1,C2,C3,C4,C5,C6,C7,C8,C9,C10,C11,C12,C13,C14,C15,C16,C17,C18,C19 >::type type;
};
}}
| {
"pile_set_name": "Github"
} |
<?xml version='1.0' encoding='UTF-8'?>
<host xmlns="urn:jboss:domain:14.0" name="master">
<extensions>
<?EXTENSIONS?>
</extensions>
<management>
<security-realms>
<security-realm name="ManagementRealm">
<authentication>
<local default-user="$local" skip-group-loading="true"/>
<properties path="mgmt-users.properties" relative-to="jboss.domain.config.dir"/>
</authentication>
<authorization map-groups-to-roles="false">
<properties path="mgmt-groups.properties" relative-to="jboss.domain.config.dir"/>
</authorization>
</security-realm>
<security-realm name="ApplicationRealm">
<server-identities>
<ssl>
<keystore path="application.keystore" relative-to="jboss.domain.config.dir" keystore-password="password" alias="server" key-password="password" generate-self-signed-certificate-host="localhost"/>
</ssl>
</server-identities>
<authentication>
<local default-user="$local" allowed-users="*" skip-group-loading="true"/>
<properties path="application-users.properties" relative-to="jboss.domain.config.dir"/>
</authentication>
<authorization>
<properties path="application-roles.properties" relative-to="jboss.domain.config.dir"/>
</authorization>
</security-realm>
</security-realms>
<audit-log>
<formatters>
<json-formatter name="json-formatter"/>
</formatters>
<handlers>
<file-handler name="host-file" formatter="json-formatter" path="audit-log.log" relative-to="jboss.domain.data.dir"/>
<file-handler name="server-file" formatter="json-formatter" path="audit-log.log" relative-to="jboss.server.data.dir"/>
</handlers>
<logger log-boot="true" log-read-only="false" enabled="false">
<handlers>
<handler name="host-file"/>
</handlers>
</logger>
<server-logger log-boot="true" log-read-only="false" enabled="false">
<handlers>
<handler name="server-file"/>
</handlers>
</server-logger>
</audit-log>
<management-interfaces>
<http-interface security-realm="ManagementRealm">
<http-upgrade enabled="true"/>
<socket interface="management" port="${jboss.management.http.port:9990}"/>
</http-interface>
</management-interfaces>
</management>
<domain-controller>
<local/>
</domain-controller>
<interfaces>
<interface name="management">
<inet-address value="${jboss.bind.address.management:127.0.0.1}"/>
</interface>
</interfaces>
<jvms>
<jvm name="default">
<heap size="64m" max-size="256m"/>
<jvm-options>
<option value="-server"/>
<option value="-XX:MetaspaceSize=96m"/>
<option value="-XX:MaxMetaspaceSize=256m"/>
</jvm-options>
</jvm>
</jvms>
<profile>
<?SUBSYSTEMS socket-binding-group="standard-sockets"?>
</profile>
</host>
| {
"pile_set_name": "Github"
} |
import React, { memo } from 'react';
import PropTypes from 'prop-types';
import { NavLink } from 'react-router-dom';
import Icon from './Icon';
function LeftMenuLink({ children, to }) {
return (
<NavLink to={to}>
<Icon />
<p>{children}</p>
</NavLink>
);
}
LeftMenuLink.defaultProps = {
children: null,
};
LeftMenuLink.propTypes = {
children: PropTypes.node,
to: PropTypes.string.isRequired,
};
export default memo(LeftMenuLink);
export { LeftMenuLink };
| {
"pile_set_name": "Github"
} |
package com.fourlastor.dante.html;
import android.content.res.Resources;
import android.graphics.Bitmap;
import android.graphics.drawable.BitmapDrawable;
import android.graphics.drawable.Drawable;
import androidx.annotation.NonNull;
public interface ImgLoader {
Drawable loadImage(@NonNull String src);
abstract class BitmapLoader implements ImgLoader {
private final Resources resources;
protected BitmapLoader(Resources resources) {
this.resources = resources;
}
@Override
public Drawable loadImage(@NonNull String src) {
Bitmap bitmap = loadBitmap(src);
BitmapDrawable bitmapDrawable = new BitmapDrawable(resources, bitmap);
bitmapDrawable.setBounds(0, 0, bitmap.getWidth(), bitmap.getHeight());
return bitmapDrawable;
}
protected abstract Bitmap loadBitmap(String src);
}
}
| {
"pile_set_name": "Github"
} |
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule invariant
*/
"use strict";
/**
* Use invariant() to assert state which your program assumes to be true.
*
* Provide sprintf-style format (only %s is supported) and arguments
* to provide information about what broke and what you were
* expecting.
*
* The invariant message will be stripped in production, but the invariant
* will remain to ensure logic does not differ in production.
*/
var invariant = function(condition, format, a, b, c, d, e, f) {
if (__DEV__) {
if (format === undefined) {
throw new Error('invariant requires an error message argument');
}
}
if (!condition) {
var error;
if (format === undefined) {
error = new Error(
'Minified exception occurred; use the non-minified dev environment ' +
'for the full error message and additional helpful warnings.'
);
} else {
var args = [a, b, c, d, e, f];
var argIndex = 0;
error = new Error(
'Invariant Violation: ' +
format.replace(/%s/g, function() { return args[argIndex++]; })
);
}
error.framesToPop = 1; // we don't care about invariant's own frame
throw error;
}
};
module.exports = invariant;
| {
"pile_set_name": "Github"
} |
Name: privateColors
Type: property
Syntax: set the privateColors to true
Summary:
Specifies whether LiveCode uses its own <color table> or the system
<color table> on <Unix|Unix systems>.
Introduced: 1.0
OS: linux
Platforms: desktop, server
Example:
set the privateColors to true
Value (bool):
The <privateColors> is true or false.
By default, the <privateColors> <property> is set to false.
Description:
Use the <privateColors> <property> to improve display on <Unix|Unix
systems> when the <bit depth> of the screen is 8 <bit|bits> (256 colors)
or less.
Set the <privateColors> <property> to true for a <stack> that uses
colors that aren't in the <default> <color table>. This has the
advantage of letting the <stack> display more colors than normally
possible on an 8- <bit> display. The disadvantage is that if the
<privateColors> is true, the colors of other applications' windows may
be distorted while LiveCode is the foreground application.
When the <privateColors> is set to false, the <engine> uses the system
<color table>. When it is set to true, the <engine> uses its own custom
<color table>.
This property has no effect unless the <screenType> <property> has a
value of "PseudoColor" --that is, each <pixel> on the screen is one of a
<color table> of colors (usually 256 colors), and the colors in that
<color table> can be changed by the <engine>.
>*Important:* Once the <privateColors> <property> is set to true, it
> cannot be set back to false. To change it back to true, you must quit
> and restart the <application>.
The setting of this property has no effect on Mac OS or Windows systems.
References: screenType (function), property (glossary),
bit depth (glossary), engine (glossary), pixel (glossary),
color table (glossary), bit (glossary), Unix (glossary),
application (glossary), default (keyword), stack (object),
remapColor (property)
| {
"pile_set_name": "Github"
} |
/**
* Mobile Photo Compress Solution
*
* Fixes iOS6 Safari's image file rendering issue for large size image (over mega-pixel),
* which causes unexpected subsampling when drawing it in canvas.
* By using this library, you can safely render the image with proper stretching.
*
* compress image in mobile browser which is file-api and canvas supported.
* be compatible with these certain situation
* 1. some android phones that do not support jpeg format output form canvas
* 2. fix ios image render in canvas
*
* Copyright (c) 2013 QZone Touch Team <tedzhou@tencent.com>
* Released under the MIT license
*/
define(function (require) {
var $ = require("zepto");
var JpegMeta = require("jpegMeta");
var JPEGEncoder = require("jpegEncoder");
var MegaPixImage = require("megapiximage");
function getImageMeta(file, callback) {
var r = new FileReader;
var err = null;
var meta = null;
r.onload = function (event) {
if (file.type === "image/jpeg") {
try {
meta = new JpegMeta.JpegFile(event.target.result, file.name)
} catch (ex) {
err = ex
}
}
callback(err, meta)
};
r.onerror = function (event) {
callback(event.target.error, meta)
};
r.readAsBinaryString(file)
}
function compress(file, picParam, callback) {
var mpImg = new MegaPixImage(file);
// defautl config
var param = $.extend({
type: "image/jpeg",
maxHeight: 800,
maxWidth: 600,
quality: .8
}, picParam);
getImageMeta(file, function (err, meta) {
// if file is a jpeg image,
// using exif messagees
// to transform the iamge at right orientation
if (meta && meta.tiff && meta.tiff.Orientation) {
param = $.extend({orientation: meta.tiff.Orientation.value}, param);
}
var canvas = document.createElement('canvas');
mpImg.onrender = function () {
var base64Str = "";
if ($.os.android && param.type == "image/jpeg") {
// using jpegEncoder to fix android machine does not support jpeg
var ctx = canvas.getContext('2d');
var imgData = ctx.getImageData(0, 0, canvas.width, canvas.height);
var encoder = new JPEGEncoder(param.quality * 100);
base64Str = encoder.encode(imgData);
encoder = null
} else {
base64Str = canvas.toDataURL(picParam.type, picParam.quality);
}
callback(base64Str);
};
mpImg.render(canvas, param);
});
}
return {compress: compress};
});
| {
"pile_set_name": "Github"
} |
//
// ZMViewController.h
// ZMBCY
//
// Created by Brance on 2017/11/24.
// Copyright © 2017年 Brance. All rights reserved.
//
#import <UIKit/UIKit.h>
#import "ZMNavView.h"
@interface ZMViewController : UIViewController
@property (nonatomic, strong) ZMNavView *navView;
- (void)setupNavView;
@end
| {
"pile_set_name": "Github"
} |