title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
💯Faster✅💯 Lesser✅4 Methods🔥Binary Search🔥Two Pointers🔥Modified Binary Search🔥Linear Search | find-first-and-last-position-of-element-in-sorted-array | 1 | 1 | # \uD83D\uDE80 Hi, I\'m [Mohammed Raziullah Ansari](https://leetcode.com/Mohammed_Raziullah_Ansari/), and I\'m excited to share 4 ways to solve this question with detailed explanation of each approach:\n\n# Problem Explaination: \nConsider a long list of numbers that are listed from smallest to largest, in that order. We refer to this list as an array. Additionally, we are looking for a particular number on this list.\n\nwe now need to know not only if that number is in the list but also when and where it first and last appears.\n\n**Here\'s an example:**\n\nArray: [1, 2, 2, 4, 4, 4, 7, 9, 10]\n\nNumber we are looking for: 4\n\nWe want to find:\n\nThe first position where the number 4 appears, which is the index 3 in this case.\nThe last position where the number 4 appears, which is the index 5 in this case.\n\n# \uD83D\uDD0D Methods To Solve This Problem:\nI\'ll be covering four different methods to solve this problem:\n1. Linear Search (Brute Force)\n2. Binary Search (Two Binary Searches)\n3. Modified Binary Search (Optimized)\n4. Two-Pointer Approach\n\n# 1. Linear Search (Brute Force): \n\n- Initialize two variables, first and last, to -1.\n- Iterate through the sorted array from left to right.\n- When you encounter the target element for the first time, set first to the current index.\n- Continue iterating to find the last occurrence of the target element and update last whenever you encounter it.\n- Return first and last\n# Complexity\n- \u23F1\uFE0F Time Complexity: O(n) - In the worst case, you may have to traverse the entire array.\n\n- \uD83D\uDE80 Space Complexity: O(1) - Constant extra space is used.\n\n# Code\n```Python []\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n first, last = -1, -1\n for i in range(len(nums)):\n if nums[i] == target:\n if first == -1:\n first = i\n last = i\n return [first, last]\n\n```\n```Java []\nclass Solution {\n public int[] searchRange(int[] nums, int target) {\n int first = -1, last = -1;\n for (int i = 0; i < nums.length; i++) {\n if (nums[i] == target) {\n if (first == -1) {\n first = i;\n }\n last = i;\n }\n }\n return new int[]{first, last};\n }\n}\n\n```\n```C++ []\nclass Solution {\npublic:\n vector<int> searchRange(vector<int>& nums, int target) {\n int first = -1, last = -1;\n for (int i = 0; i < nums.size(); ++i) {\n if (nums[i] == target) {\n if (first == -1) {\n first = i;\n }\n last = i;\n }\n }\n return {first, last};\n }\n};\n\n```\n```C []\nint* searchRange(int* nums, int numsSize, int target, int* returnSize) {\n int first = -1, last = -1;\n for (int i = 0; i < numsSize; i++) {\n if (nums[i] == target) {\n if (first == -1) {\n first = i;\n }\n last = i;\n }\n }\n\n int* result = (int*)malloc(2 * sizeof(int));\n result[0] = first;\n result[1] = last;\n *returnSize = 2;\n return result;\n}\n\n```\n# 2. Binary Search (Two Binary Searches):\n- Perform a binary search to find the first occurrence of the target element.\n- Perform another binary search to find the last occurrence of the target element.\n- Return the results from the two binary searches.\n# Complexity\n- \u23F1\uFE0F Time Complexity: O(n) - Two binary searches are performed.\n\n- \uD83D\uDE80 Space Complexity: O(1) - Constant extra space is used.\n\n# Code\n```Python []\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n def findFirst(nums, target):\n left, right = 0, len(nums) - 1\n while left <= right:\n mid = left + (right - left) // 2\n if nums[mid] == target:\n if mid == 0 or nums[mid - 1] != target:\n return mid\n else:\n right = mid - 1\n elif nums[mid] < target:\n left = mid + 1\n else:\n right = mid - 1\n return -1\n \n def findLast(nums, target):\n left, right = 0, len(nums) - 1\n while left <= right:\n mid = left + (right - left) // 2\n if nums[mid] == target:\n if mid == len(nums) - 1 or nums[mid + 1] != target:\n return mid\n else:\n left = mid + 1\n elif nums[mid] < target:\n left = mid + 1\n else:\n right = mid - 1\n return -1\n \n first = findFirst(nums, target)\n last = findLast(nums, target)\n return [first, last]\n\n```\n```Java []\nclass Solution {\n public int[] searchRange(int[] nums, int target) {\n int first = findFirst(nums, target);\n int last = findLast(nums, target);\n return new int[]{first, last};\n }\n\n private int findFirst(int[] nums, int target) {\n int left = 0, right = nums.length - 1;\n int first = -1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n if (mid == 0 || nums[mid - 1] != target) {\n first = mid;\n break;\n } else {\n right = mid - 1;\n }\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n return first;\n }\n\n private int findLast(int[] nums, int target) {\n int left = 0, right = nums.length - 1;\n int last = -1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n if (mid == nums.length - 1 || nums[mid + 1] != target) {\n last = mid;\n break;\n } else {\n left = mid + 1;\n }\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n return last;\n }\n}\n\n```\n```C++ []\nclass Solution {\npublic:\n vector<int> searchRange(vector<int>& nums, int target) {\n int first = findFirst(nums, target);\n int last = findLast(nums, target);\n return {first, last};\n }\n\nprivate:\n int findFirst(vector<int>& nums, int target) {\n int left = 0, right = nums.size() - 1;\n int first = -1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n if (mid == 0 || nums[mid - 1] != target) {\n first = mid;\n break;\n }\n else {\n right = mid - 1;\n }\n }\n else if (nums[mid] < target) {\n left = mid + 1;\n }\n else {\n right = mid - 1;\n }\n }\n return first;\n }\n\n int findLast(vector<int>& nums, int target) {\n int left = 0, right = nums.size() - 1;\n int last = -1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n if (mid == nums.size() - 1 || nums[mid + 1] != target) {\n last = mid;\n break;\n }\n else {\n left = mid + 1;\n }\n }\n else if (nums[mid] < target) {\n left = mid + 1;\n }\n else {\n right = mid - 1;\n }\n }\n return last;\n }\n};\n\n```\n```C []\nint* searchRange(int* nums, int numsSize, int target, int* returnSize) {\n int first = findFirst(nums, numsSize, target);\n int last = findLast(nums, numsSize, target);\n\n int* result = (int*)malloc(2 * sizeof(int));\n result[0] = first;\n result[1] = last;\n *returnSize = 2;\n return result;\n}\n\nint findFirst(int* nums, int numsSize, int target) {\n int left = 0, right = numsSize - 1;\n int first = -1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n if (mid == 0 || nums[mid - 1] != target) {\n first = mid;\n break;\n } else {\n right = mid - 1;\n }\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n return first;\n}\n\nint findLast(int* nums, int numsSize, int target) {\n int left = 0, right = numsSize - 1;\n int last = -1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n if (mid == numsSize - 1 || nums[mid + 1] != target) {\n last = mid;\n break;\n } else {\n left = mid + 1;\n }\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n return last;\n}\n\n```\n# 3. Modified Binary Search (Optimized):\n - Initialize two variables, first and last, to -1.\n- Perform a binary search to find the target element.\n- When you find the target element, update first to the current index and continue searching for the first occurrence in the left subarray.\n- Similarly, update last to the current index and continue searching for the last occurrence in the right subarray.\n- Continue until the binary search terminates.\n- Return first and last.\n# Complexity\n- \u23F1\uFE0F Time Complexity: O(n) - One binary search with constant additional work.\n\n- \uD83D\uDE80 Space Complexity: O(1) - Constant extra space is used.\n\n# Code\n```Python []\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n def findFirst(nums, target):\n left, right = 0, len(nums) - 1\n first = -1\n while left <= right:\n mid = left + (right - left) // 2\n if nums[mid] == target:\n first = mid\n right = mid - 1\n elif nums[mid] < target:\n left = mid + 1\n else:\n right = mid - 1\n return first\n \n def findLast(nums, target):\n left, right = 0, len(nums) - 1\n last = -1\n while left <= right:\n mid = left + (right - left) // 2\n if nums[mid] == target:\n last = mid\n left = mid + 1\n elif nums[mid] < target:\n left = mid + 1\n else:\n right = mid - 1\n return last\n \n first = findFirst(nums, target)\n last = findLast(nums, target)\n return [first, last]\n\n```\n```Java []\nclass Solution {\n public int[] searchRange(int[] nums, int target) {\n int first = findFirst(nums, target);\n int last = findLast(nums, target);\n return new int[]{first, last};\n }\n\n private int findFirst(int[] nums, int target) {\n int left = 0, right = nums.length - 1;\n int first = -1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n first = mid;\n right = mid - 1;\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n return first;\n }\n\n private int findLast(int[] nums, int target) {\n int left = 0, right = nums.length - 1;\n int last = -1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n last = mid;\n left = mid + 1;\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n return last;\n }\n}\n\n```\n```C++ []\nclass Solution {\npublic:\n vector<int> searchRange(vector<int>& nums, int target) {\n int first = findFirst(nums, target);\n int last = findLast(nums, target);\n return {first, last};\n }\n\nprivate:\n int findFirst(vector<int>& nums, int target) {\n int left = 0, right = nums.size() - 1;\n int first = -1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n first = mid;\n right = mid - 1;\n }\n else if (nums[mid] < target) {\n left = mid + 1;\n }\n else {\n right = mid - 1;\n }\n }\n return first;\n }\n\n int findLast(vector<int>& nums, int target) {\n int left = 0, right = nums.size() - 1;\n int last = -1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n last = mid;\n left = mid + 1;\n }\n else if (nums[mid] < target) {\n left = mid + 1;\n }\n else {\n right = mid - 1;\n }\n }\n return last;\n }\n};\n\n```\n```C []\nint* searchRange(int* nums, int numsSize, int target, int* returnSize) {\n int first = findFirst(nums, numsSize, target);\n int last = findLast(nums, numsSize, target);\n\n int* result = (int*)malloc(2 * sizeof(int));\n result[0] = first;\n result[1] = last;\n *returnSize = 2;\n return result;\n}\n\nint findFirst(int* nums, int numsSize, int target) {\n int left = 0, right = numsSize - 1;\n int first = -1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n first = mid;\n right = mid - 1;\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n return first;\n}\n\nint findLast(int* nums, int numsSize, int target) {\n int left = 0, right = numsSize - 1;\n int last = -1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n last = mid;\n left = mid + 1;\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n return last;\n}\n\n```\n# 4. Two-Pointer Approach:\n - Initialize two pointers, left and right, to the beginning and end of the array, respectively.\n- Initialize two variables, first and last, to -1.\n- Use a while loop with the condition left <= right.\n- Calculate the middle index as (left + right) / 2.\n- If the middle element is equal to the target, update first and last accordingly and adjust the pointers.\n- If the middle element is less than the target, update left.\n- If the middle element is greater than the target, update right.\n- Continue the loop until left is less than or equal to right.\n- Return first and last.\n# Complexity\n- \u23F1\uFE0F Time Complexity: O(n) - One pass through the array using two pointers.\n\n- \uD83D\uDE80 Space Complexity: O(1) - Constant extra space is used.\n\n# Code\n```Python []\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n left, right = 0, len(nums) - 1\n first, last = -1, -1\n \n while left <= right:\n mid = left + (right - left) // 2\n if nums[mid] == target:\n first = mid\n last = mid\n while first > 0 and nums[first - 1] == target:\n first -= 1\n while last < len(nums) - 1 and nums[last + 1] == target:\n last += 1\n return [first, last]\n elif nums[mid] < target:\n left = mid + 1\n else:\n right = mid - 1\n \n return [first, last]\n\n```\n```Java []\nclass Solution {\n public int[] searchRange(int[] nums, int target) {\n int left = 0, right = nums.length - 1;\n int first = -1, last = -1;\n\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n first = mid;\n last = mid;\n while (first > 0 && nums[first - 1] == target) {\n first--;\n }\n while (last < nums.length - 1 && nums[last + 1] == target) {\n last++;\n }\n break;\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n\n return new int[]{first, last};\n }\n}\n\n```\n```C++ []\nclass Solution {\npublic:\n vector<int> searchRange(vector<int>& nums, int target) {\n int left = 0, right = nums.size() - 1;\n int first = -1, last = -1;\n \n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n first = mid;\n last = mid;\n while (first > 0 && nums[first - 1] == target) {\n first--;\n }\n while (last < nums.size() - 1 && nums[last + 1] == target) {\n last++;\n }\n break;\n }\n else if (nums[mid] < target) {\n left = mid + 1;\n }\n else {\n right = mid - 1;\n }\n }\n \n return {first, last};\n }\n};\n\n```\n```C []\nint* searchRange(int* nums, int numsSize, int target, int* returnSize) {\n int left = 0, right = numsSize - 1;\n int first = -1, last = -1;\n\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] == target) {\n first = mid;\n last = mid;\n while (first > 0 && nums[first - 1] == target) {\n first--;\n }\n while (last < numsSize - 1 && nums[last + 1] == target) {\n last++;\n }\n break;\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n\n int* result = (int*)malloc(2 * sizeof(int));\n result[0] = first;\n result[1] = last;\n *returnSize = 2;\n return result;\n}\n\n```\n# \uD83C\uDFC6Conclusion: \nThe most effective methods are Methods 3 and 4, which have constant space complexity and an O(n) time complexity. \n\n\n# \uD83D\uDCA1 I invite you to check out my profile for detailed explanations and code for each method. Happy coding and learning! \uD83D\uDCDA | 88 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Python3 Solution | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | \n```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n n=len(nums)\n left=0\n right=n-1\n if target in nums:\n for i in range(0,n):\n if nums[left]==nums[right]:\n return [left,right] \n elif nums[left]<target:\n left+=1\n else:\n right-=1\n else:\n return [-1,-1] \n``` | 2 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Python solution easy to understand with optimise space and time complexity | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | If you like the solution please give \u2B50 to me\n# Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe code aims to find the starting and ending positions of a target element in a sorted array using a linear search approach.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nInitialize two pointers, left and right, to the beginning and end of the array, respectively.\nUse a while loop to iterate from left to the end of the array to find the leftmost occurrence of the target element.\nUse another while loop to iterate from right to the beginning of the array to find the rightmost occurrence of the target element.\nStore the indices of the leftmost and rightmost occurrences in the list l.\nIf no occurrences are found (length of l is 0), return [-1, -1]. Otherwise, return the list l.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n# Code\n```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n left = 0\n right = len(nums)-1\n l = []\n while left <len(nums):\n if nums[left]==target:\n l.append(left)\n break\n left +=1\n while right >=0:\n if nums[right]==target:\n l.append(right)\n break\n right -=1 \n if len(l) ==0:\n return [-1,-1]\n else:\n return l \n``` | 1 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
100% BEATS - BEGINNER FRIENDLY CODE IN BINARY SEARCH (TIME - O(LOG N) ,SPACE - O(1)) | find-first-and-last-position-of-element-in-sorted-array | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThis code defines a Solution with three member functions: search_first, search_last, and searchRange. The searchRange function aims to find the first and last occurrence of a target value in a sorted array of integers.\n\nHere\'s a breakdown of each function\'s purpose and logic:\n\n search_first function:\n Input Parameters:\n nums: A vector of integers representing a sorted array.\n target: The value to search for in the array.\n n: The size of the array nums.\n Output:\n Returns the index of the first occurrence of the target in the array, or -1 if the target is not present.\n Algorithm:\n Uses binary search to find the first occurrence of the target in the array by modifying the search range based on whether the current element is equal to, greater than, or less than the target.\n\n search_last function:\n Input Parameters:\n nums: A vector of integers representing a sorted array.\n target: The value to search for in the array.\n n: The size of the array nums.\n Output:\n Returns the index of the last occurrence of the target in the array, or -1 if the target is not present.\n Algorithm:\n Uses binary search to find the last occurrence of the target in the array by modifying the search range based on whether the current element is equal to, greater than, or less than the target.\n\n searchRange function:\n Input Parameters:\n nums: A vector of integers representing a sorted array.\n target: The value to search for in the array.\n Output:\n Returns a vector containing two elements: the index of the first occurrence of the target and the index of the last occurrence of the target.\n Algorithm:\n Calls search_first and search_last to find the first and last occurrence of the target in the array and returns a vector with these indices.\n\nOverall, the searchRange function uses binary search to find the first and last occurrence of the target in the sorted array and returns this information in a vector.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe provided code implements a binary search-based approach to find the first and last occurrences of a target value in a sorted array. Let\'s discuss the approach in detail for both the search_first and search_last functions.\nsearch_first Function:\n\nThe purpose of this function is to find the index of the first occurrence of the target value in the sorted array.\n\n Initialize Variables:\n Set low to the beginning of the array (0).\n Set high to the end of the array (n - 1).\n Set ans to -1, which will store the index of the first occurrence.\n\n Binary Search:\n While low is less than or equal to high, perform the following:\n Calculate the mid index as (low + high) / 2.\n If the element at the mid index equals the target:\n Update ans to the mid index.\n Move high to mid - 1 to search for the first occurrence to the left.\n If the element at the mid index is greater than the target:\n Update high to mid - 1 to search in the left half.\n If the element at the mid index is less than the target:\n Update low to mid + 1 to search in the right half.\n\n Return Result:\n Return ans, which contains the index of the first occurrence of the target, or -1 if the target is not found.\n\nsearch_last Function:\n\nThe purpose of this function is to find the index of the last occurrence of the target value in the sorted array.\n\n Initialize Variables:\n Set low to the beginning of the array (0).\n Set high to the end of the array (n - 1).\n Set ans to -1, which will store the index of the last occurrence.\n\n Binary Search:\n While low is less than or equal to high, perform the following:\n Calculate the mid index as (low + high) / 2.\n If the element at the mid index equals the target:\n Update ans to the mid index.\n Move low to mid + 1 to search for the last occurrence to the right.\n If the element at the mid index is less than the target:\n Update low to mid + 1 to search in the right half.\n If the element at the mid index is greater than the target:\n Update high to mid - 1 to search in the left half.\n\n Return Result:\n Return ans, which contains the index of the last occurrence of the target, or -1 if the target is not found.\n\nsearchRange Function:\n\nThe searchRange function uses the search_first and search_last functions to find the first and last occurrences of the target in the sorted array. It then returns a vector containing these indices as the result.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(log N)\nLet\'s analyze the time and space complexity of the provided code for both the search_first and search_last functions, as well as the overall searchRange function.\nTime Complexity:\n\n search_first Function:\n The time complexity of the binary search algorithm is O(log n) since at each step, the search range is halved.\n\n search_last Function:\n The time complexity of the binary search algorithm is O(log n) since at each step, the search range is halved.\n\n searchRange Function:\n Calls to search_first and search_last functions both have a time complexity of O(log n) since they use binary search.\n\nOverall Time Complexity:\n\nSince both search_first and search_last functions are called within the searchRange function, and both have a time complexity of O(log n), the overall time complexity of the searchRange function is also O(log n).\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\nThe space complexity of the provided code is determined by the space used by the input vector nums and the additional variables used within each function. However, the additional variables used within the functions have a constant space requirement and do not depend on the input size.\n\nTherefore, the space complexity is primarily determined by the input vector nums, which is passed by reference and does not incur additional space complexity. Hence, the space complexity of the code is O(1), constant space.\n\n# Code\n```\nclass Solution {\npublic:\n int search_first(vector<int>& nums, int target,int n){\n int low = 0;\n int high = n-1;\n int ans = -1;\n while(low <= high){\n int mid = (low+high)/2;\n if(nums[mid] == target) {\n ans = mid;\n high = mid-1;\n }\n else if(nums[mid] > target) high = mid-1;\n else low = mid+1;\n }\n return ans;\n }\n\n int search_last(vector<int>& nums, int target,int n){\n int low = 0;\n int high = n-1;\n int ans = -1;\n while(low <= high){\n int mid = (low+high)/2;\n if(nums[mid] == target){\n ans = mid;\n low = mid+1;\n }\n else if(nums[mid] < target) low = mid+1;\n else high = mid-1;\n }\n return ans;\n }\n\n vector<int> searchRange(vector<int>& nums, int target) {\n vector<int> ans;\n int n = nums.size();\n int first = search_first(nums,target,n);\n int last = search_last(nums,target,n);\n ans.push_back(first);\n ans.push_back(last);\n return ans;\n }\n};\n```\n```c++ []\nlass Solution {\npublic:\n int search_first(vector<int>& nums, int target,int n){\n int low = 0;\n int high = n-1;\n int ans = -1;\n while(low <= high){\n int mid = (low+high)/2;\n if(nums[mid] == target) {\n ans = mid;\n high = mid-1;\n }\n else if(nums[mid] > target) high = mid-1;\n else low = mid+1;\n }\n return ans;\n }\n\n int search_last(vector<int>& nums, int target,int n){\n int low = 0;\n int high = n-1;\n int ans = -1;\n while(low <= high){\n int mid = (low+high)/2;\n if(nums[mid] == target){\n ans = mid;\n low = mid+1;\n }\n else if(nums[mid] < target) low = mid+1;\n else high = mid-1;\n }\n return ans;\n }\n\n vector<int> searchRange(vector<int>& nums, int target) {\n vector<int> ans;\n int n = nums.size();\n int first = search_first(nums,target,n);\n int last = search_last(nums,target,n);\n ans.push_back(first);\n ans.push_back(last);\n return ans;\n }\n};\n```\n```java []\nimport java.util.ArrayList;\nimport java.util.List;\n\nclass Solution {\n public int search_first(List<Integer> nums, int target, int n) {\n int low = 0;\n int high = n - 1;\n int ans = -1;\n while (low <= high) {\n int mid = (low + high) / 2;\n if (nums.get(mid) == target) {\n ans = mid;\n high = mid - 1;\n } else if (nums.get(mid) > target) {\n high = mid - 1;\n } else {\n low = mid + 1;\n }\n }\n return ans;\n }\n\n public int search_last(List<Integer> nums, int target, int n) {\n int low = 0;\n int high = n - 1;\n int ans = -1;\n while (low <= high) {\n int mid = (low + high) / 2;\n if (nums.get(mid) == target) {\n ans = mid;\n low = mid + 1;\n } else if (nums.get(mid) < target) {\n low = mid + 1;\n } else {\n high = mid - 1;\n }\n }\n return ans;\n }\n\n public int[] searchRange(int[] nums, int target) {\n List<Integer> ans = new ArrayList<>();\n int n = nums.size();\n int first = search_first(nums, target, n);\n int last = search_last(nums, target, n);\n ans.add(first);\n ans.add(last);\n return ans;\n }\n}\n```\n```python []\nclass Solution(object):\n def searchRange(self,nums, target):\n def search_first(nums, target, n):\n low = 0\n high = n-1\n ans = -1\n while low <= high:\n mid = (low+high)//2\n if nums[mid] == target:\n ans = mid\n high = mid-1\n elif nums[mid] > target:\n high = mid-1\n else:\n low = mid+1\n return ans\n\n def search_last(nums, target, n):\n low = 0\n high = n-1\n ans = -1\n while low <= high:\n mid = (low+high)//2\n if nums[mid] == target:\n ans = mid\n low = mid+1\n elif nums[mid] < target:\n low = mid+1\n else:\n high = mid-1\n return ans \n\n\n ans = []\n n = len(nums)\n first = search_first(nums, target, n)\n last = search_last(nums, target, n)\n ans.append(first)\n ans.append(last)\n return ans\n```\n``` python3 []\n class Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n def search_first(nums, target, n):\n low = 0\n high = n-1\n ans = -1\n while low <= high:\n mid = (low+high)//2\n if nums[mid] == target:\n ans = mid\n high = mid-1\n elif nums[mid] > target:\n high = mid-1\n else:\n low = mid+1\n return ans\n\n def search_last(nums, target, n):\n low = 0\n high = n-1\n ans = -1\n while low <= high:\n mid = (low+high)//2\n if nums[mid] == target:\n ans = mid\n low = mid+1\n elif nums[mid] < target:\n low = mid+1\n else:\n high = mid-1\n return ans \n\n\n ans = []\n n = len(nums)\n first = search_first(nums, target, n)\n last = search_last(nums, target, n)\n ans.append(first)\n ans.append(last)\n return ans\n```\n# **PLEASE UPVOTE THIS CODE***\n | 1 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
✅ 3 ms || Intuitive solution || C++ || 2 methods || Binary Search | find-first-and-last-position-of-element-in-sorted-array | 1 | 1 | # Intuition\n\nWe have to find elements in $$O(logn)$$ time complexity, So the first thing that comes into mind is Binary Search!\n\n# Approach\n\n1. The `search` function is used to find the insertion point of the target value in the sorted vector. It returns the index where the target value should be inserted or the index of the first occurrence of a value greater than the target value.\n\n2. The `searchRange` function initializes `l` and `r` as the left and right boundaries of the input vector, respectively.\n\n3. It uses the `search` function to find the starting position `str` of the target value and the ending position `end` of the next value (target value + 1).\n\n4. If `str` is within the bounds of the vector and the value at `ar[str]` is equal to the target value, it means the target value is present, and the function returns the range [str, end-1].\n\n5. If the target value is not found, the function returns [-1, -1].\n\n# Complexity\n- Time complexity: $$O(log n)$$ for the binary search, where n is the size of the input vector.\n- Space complexity: $$O(1)$$ as the code uses a constant amount of extra space.\n\n```cpp []\n//Method 1\nclass Solution {\npublic:\n int search(vector<int> &ar, int l, int r, int tg) {\n while (l <= r) {\n int mid = (r + l) / 2;\n if (ar[mid] < tg) {\n l = mid + 1;\n } else {\n r = mid - 1;\n }\n }\n return l;\n }\n\n vector<int> searchRange(vector<int>& ar, int tg) {\n int l = 0, r = ar.size() - 1;\n int str = search(ar, l, r, tg);\n int end = search(ar, l, r, tg + 1) - 1;\n\n if (str < ar.size() && ar[str] == tg) {\n return {str, end};\n }\n\n return {-1, -1};\n }\n};\n```\n```cpp []\n//#Method 2\nclass Solution {\npublic:\n std::vector<int> searchRange(std::vector<int>& nums, int target) {\n int first = findFirst(nums, target);\n int last = findLast(nums, target);\n \n if (first != -1 && last != -1) {\n return {first, last};\n } else {\n return {-1, -1};\n }\n }\n \nprivate:\n int findFirst(std::vector<int>& nums, int target) {\n int left = 0;\n int right = nums.size() - 1;\n int result = -1;\n \n while (left <= right) {\n int mid = left + (right - left) / 2;\n \n if (nums[mid] >= target) {\n right = mid - 1;\n } else {\n left = mid + 1;\n }\n \n if (nums[mid] == target) {\n result = mid; // Update result whenever target is found.\n }\n }\n \n return result;\n }\n \n int findLast(std::vector<int>& nums, int target) {\n int left = 0;\n int right = nums.size() - 1;\n int result = -1;\n \n while (left <= right) {\n int mid = left + (right - left) / 2;\n \n if (nums[mid] <= target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n \n if (nums[mid] == target) {\n result = mid; // Update result whenever target is found.\n }\n }\n \n return result;\n }\n};\n\n```\n```python []\nfrom typing import List\n\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n first = self.findFirst(nums, target)\n last = self.findLast(nums, target)\n \n if first != -1 and last != -1:\n return [first, last]\n else:\n return [-1, -1]\n \n def findFirst(self, nums: List[int], target: int) -> int:\n left, right = 0, len(nums) - 1\n result = -1\n \n while left <= right:\n mid = left + (right - left) // 2\n \n if nums[mid] >= target:\n right = mid - 1\n else:\n left = mid + 1\n \n if nums[mid] == target:\n result = mid # Update result whenever target is found.\n \n return result\n \n def findLast(self, nums: List[int], target: int) -> int:\n left, right = 0, len(nums) - 1\n result = -1\n \n while left <= right:\n mid = left + (right - left) // 2\n \n if nums[mid] <= target:\n left = mid + 1\n else:\n right = mid - 1\n \n if nums[mid] == target:\n result = mid # Update result whenever target is found.\n \n return result\n```\n```Java []\nimport java.util.Arrays;\n\nclass Solution {\n public int[] searchRange(int[] nums, int target) {\n int first = findFirst(nums, target);\n int last = findLast(nums, target);\n \n if (first != -1 && last != -1) {\n return new int[]{first, last};\n } else {\n return new int[]{-1, -1};\n }\n }\n \n private int findFirst(int[] nums, int target) {\n int left = 0;\n int right = nums.length - 1;\n int result = -1;\n \n while (left <= right) {\n int mid = left + (right - left) / 2;\n \n if (nums[mid] >= target) {\n right = mid - 1;\n } else {\n left = mid + 1;\n }\n \n if (nums[mid] == target) {\n result = mid; // Update result whenever target is found.\n }\n }\n \n return result;\n }\n \n private int findLast(int[] nums, int target) {\n int left = 0;\n int right = nums.length - 1;\n int result = -1;\n \n while (left <= right) {\n int mid = left + (right - left) / 2;\n \n if (nums[mid] <= target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n \n if (nums[mid] == target) {\n result = mid; // Update result whenever target is found.\n }\n }\n \n return result;\n }\n}\n\n```\n\n | 1 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
✅✅✅Easy, understandable Python solution | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nwe have to apply binary search twice to find the 2 ends of the subarray filled with target\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nIt first searches for the left index where the target is found and then for the right index. If both indices are valid (left <= right), it returns them as the range of indices where the target appears in the list. If the target is not found, it returns [-1, -1]\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(logn)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n\n# Code\n```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n if(nums == None or len(nums) == 0):\n return [-1,-1]\n n = len(nums)\n \n def findFirst():\n left = 0\n right = n-1\n while(left<=right):\n mid = left + (right-left)//2\n if(nums[mid]>=target):\n right = mid-1\n else:\n left = mid + 1\n return left\n def findLast():\n left = 0\n right = n-1\n while(left<=right):\n mid = left + (right-left)//2\n if(nums[mid]<=target):\n left = mid + 1\n else:\n right = mid - 1\n return right\n first = findFirst()\n last = findLast()\n if(first > n-1 or first < 0 or nums[first] != target):\n return [-1,-1]\n return [first, last]\n``` | 1 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
python3 code | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | # Intuition\nThis code defines a function called searchRange that takes in a list of integers (nums) and a target integer (target) as inputs. The function returns a list containing the starting and ending index of the target integer in the input list. If the target integer is not present in the list, the function returns [-1, -1].\n\nThe function first initializes an empty list called l and counts the number of times the target integer appears in the input list using the count() method. If the target integer is present in the input list, the function uses the index() method to find the starting index of the target integer and calculates the ending index by adding the count of the target integer minus 1 to the starting index. The starting and ending indices are then appended to the l list. If the target integer is not present in the input list, [-1, -1] is appended to the l list. Finally, the function returns the l list.\n\n# Approach\nThe approach of the code is to find the starting and ending index of a target integer in a given list of integers. It first checks if the target integer is present in the list using the in operator. If it is present, it uses the index() method to find the starting index of the target integer and calculates the ending index by adding the count of the target integer minus 1 to the starting index. The starting and ending indices are then appended to a list. If the target integer is not present in the list, [-1, -1] is appended to the list. Finally, the function returns the list containing the starting and ending indices of the target integer or [-1, -1] if the target integer is not present in the list.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n l=[]\n c = nums.count(target)\n\n if target in nums:\n x = nums.index(target)\n y = x+c-1\n l.append(x)\n l.append(y)\n else:\n l.append(-1)\n l.append(-1)\n \n return l\n \n``` | 1 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Python code for finding the starting and ending position of a given target value | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | # Intuition\nIt first checks if the target exists in the nums list using the in operator. If not, it returns [-1, -1] to indicate that the target is not present.\n\nIf the target is found in nums, it calculates the starting index by using the index() method to find the first occurrence of the target. It calculates the ending index by reversing the list and again using the index() method to find the last occurrence of the target.\n\nThe code returns a list containing the starting and ending indices of the target number within nums.\n\n\n# Approach\nThe code\'s key idea is to use the index() method to find the first and \n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution(object):\n def searchRange(self, nums, target):\n if target in nums:\n return [nums.index(target),(len(nums)-1)-nums[::-1].index(target)]\n else:\n return [-1,-1]\n \n``` | 1 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
🤯 CRAZY ONE-LINE SOLUTION IN PYTHON | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | # Approach\nUse `bisect_left` and `bisect_right` to find leftmost and rightmost indexes.\n\nIf the leftmost index gets out of range or there is no target in array, then return `[-1, -1]`.\n\nElse return `[leftmost, rightmost - 1]`\n\n# Complexity\n- Time complexity: $$O(\\log n)$$\n- Space complexity: $$O(\\log n)$$\n\n# Code\n```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n return [-1, -1] if (first := bisect_left(nums, target)) >= len(nums) or nums[first] != target else [first, bisect_right(nums, target) - 1]\n```\n\n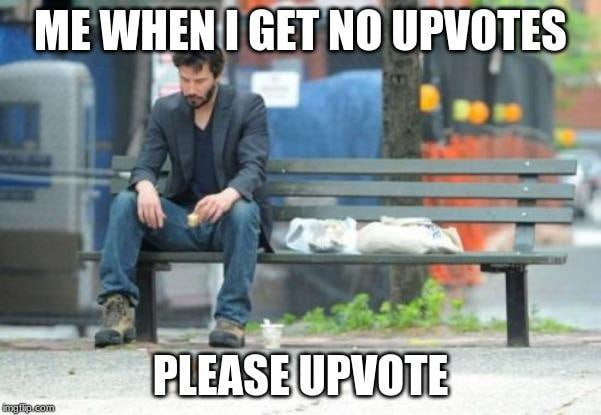\n | 3 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
【Video】Give me 10 minutes - How we think about a solution - Binary Search | find-first-and-last-position-of-element-in-sorted-array | 1 | 1 | Welcome to my post! This post starts with "How we think about a solution". In other words, that is my thought process to solve the question. This post explains how I get to my solution instead of just posting solution codes or out of blue algorithms. I hope it is helpful for someone.\n\n# Intuition\nUsing Binary Search twice for left most value and right most value\n\n---\n\n# Solution Video\n\nhttps://youtu.be/441pamgku74\n\n\u25A0 Timeline of the video\n`0:00` Read the question of Find First and Last Position of Element in Sorted Array\n`1:16` Demonstrate solution with an example\n`6:59` Coding\n`9:56` Time Complexity and Space Complexity\n`10:14` Step by step algorithm\n\nI created the video last year. I write my thought process in the post to support the video. You can understand the question visually with the video and behind the scene with the post.\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 2,637\nMy initial goal is 10,000\nThank you for your support!\n\n---\n\n# Approach\n\n## How we think about a solution\n\nWe have a constraint of time complexity $$O(log n)$$ and a description says "Given an array of integers `nums` sorted in non-decreasing order", so we can feel this question is solved by `binary search`.\n\nBut a challenging point of this question is that we might multiple targes in the input array, becuase we have to find the starting and ending position of a given target value.\n\n\n---\n\n\u2B50\uFE0F Points\n\nWe might have multiple targets in the input array.\n\n---\n\nThe start position and end position can be rephrased as the leftmost index and the rightmost index.\n\nSo, for the most part, we use typical binary search, but when we find a target, we need to do these.\n\n---\n\n\u2B50\uFE0F Points\n\nEvery time we find one of targets, `keep current index` and execute one of these\n\n- If we try to find left most index, move `right pointer` to `middle pointer - 1`.\n- If we try to find right most index, move `left pointer` to `middle pointer + 1`.\n\n\nBecause we need to find a target again between `left and middle pointer` or `right and middle pointer`. If we don\'t find the new target in the new range, don\'t worry. The current index you have right now is the most left index or right index.\n\n---\n\n- How we can find the most left index and right index at the same time?\n\nI think it\'s hard to find the most left index and right index at the same time, so simply we execute binary search twice for the most left index and right index, so that we can focus on one of them.\n\nLet\'s see a real algorithm!\n\n\n### Algorithm Overview:\n\n1. Define a class `Solution`.\n2. Implement a method `searchRange` that takes a sorted list of integers `nums` and a target integer `target`. This method finds the range of the target value in the input list.\n\n### Detailed Explanation:\n1. Define a class named `Solution`.\n2. Implement a method `searchRange` within the `Solution` class that takes three parameters: `nums` (a sorted list of integers), `target` (an integer to search for), and `is_searching_left` (a boolean indicating whether to search for the leftmost or rightmost occurrence of the target).\n\n a. Define a helper method `binary_search` that takes `nums`, `target`, and `is_searching_left` as arguments. This method performs binary search to find the target value and returns the index of either the leftmost or rightmost occurrence of the target value based on the `is_searching_left` parameter.\n\n b. Initialize `left` to 0, `right` to the length of `nums` minus 1, and `idx` to -1.\n\n c. Perform a binary search within the `nums` array using a while loop until `left` is less than or equal to `right`.\n\n d. Calculate the midpoint as `(left + right) // 2`.\n\n e. Compare the target value with the element at the midpoint of the array (`nums[mid]`):\n - If `nums[mid]` is greater than the target, update `right` to `mid - 1`.\n - If `nums[mid]` is less than the target, update `left` to `mid + 1`.\n - If `nums[mid]` is equal to the target, update `idx` to `mid` and adjust `left` or `right` accordingly based on `is_searching_left`.\n\n f. Return the index `idx`.\n\n3. Call `binary_search` twice within the `searchRange` method: once to find the leftmost occurrence of the target (`left = binary_search(nums, target, True)`) and once to find the rightmost occurrence of the target (`right = binary_search(nums, target, False)`).\n\n4. Return a list containing the leftmost and rightmost indices of the target: `[left, right]`.\n\nThis algorithm uses binary search to efficiently find the leftmost and rightmost occurrences of the target in the sorted array.\n\n# Complexity\n- Time complexity: $$O(log n)$$\nThe time complexity of the binary search algorithm is $$O(log n)$$ where n is the length of the input array nums. The searchRange method calls binary_search twice, so the overall time complexity remains $$O(log n)$$.\n\n- Space complexity: $$O(1)$$\nThe space complexity is $$O(1)$$ because the algorithm uses a constant amount of extra space, regardless of the size of the input array. There are no data structures or recursive calls that consume additional space proportional to the input size.\n\n```python []\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n \n def binary_search(nums, target, is_searching_left):\n left = 0\n right = len(nums) - 1\n idx = -1\n \n while left <= right:\n mid = (left + right) // 2\n \n if nums[mid] > target:\n right = mid - 1\n elif nums[mid] < target:\n left = mid + 1\n else:\n idx = mid\n if is_searching_left:\n right = mid - 1\n else:\n left = mid + 1\n \n return idx\n \n left = binary_search(nums, target, True)\n right = binary_search(nums, target, False)\n \n return [left, right]\n \n```\n```javascript []\n/**\n * @param {number[]} nums\n * @param {number} target\n * @return {number[]}\n */\nvar searchRange = function(nums, target) {\n const binarySearch = (nums, target, isSearchingLeft) => {\n let left = 0;\n let right = nums.length - 1;\n let idx = -1;\n \n while (left <= right) {\n const mid = Math.floor((left + right) / 2);\n \n if (nums[mid] > target) {\n right = mid - 1;\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n idx = mid;\n if (isSearchingLeft) {\n right = mid - 1;\n } else {\n left = mid + 1;\n }\n }\n }\n \n return idx;\n };\n \n const left = binarySearch(nums, target, true);\n const right = binarySearch(nums, target, false);\n \n return [left, right]; \n};\n```\n```java []\nclass Solution {\n public int[] searchRange(int[] nums, int target) {\n int[] result = {-1, -1};\n int left = binarySearch(nums, target, true);\n int right = binarySearch(nums, target, false);\n result[0] = left;\n result[1] = right;\n return result; \n }\n\n private int binarySearch(int[] nums, int target, boolean isSearchingLeft) {\n int left = 0;\n int right = nums.length - 1;\n int idx = -1;\n\n while (left <= right) {\n int mid = left + (right - left) / 2;\n \n if (nums[mid] > target) {\n right = mid - 1;\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n idx = mid;\n if (isSearchingLeft) {\n right = mid - 1;\n } else {\n left = mid + 1;\n }\n }\n }\n\n return idx;\n }\n\n}\n```\n```C++ []\nclass Solution {\npublic:\n vector<int> searchRange(vector<int>& nums, int target) {\n vector<int> result = {-1, -1};\n int left = binarySearch(nums, target, true);\n int right = binarySearch(nums, target, false);\n result[0] = left;\n result[1] = right;\n return result; \n }\n\n int binarySearch(vector<int>& nums, int target, bool isSearchingLeft) {\n int left = 0;\n int right = nums.size() - 1;\n int idx = -1;\n \n while (left <= right) {\n int mid = left + (right - left) / 2;\n \n if (nums[mid] > target) {\n right = mid - 1;\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n idx = mid;\n if (isSearchingLeft) {\n right = mid - 1;\n } else {\n left = mid + 1;\n }\n }\n }\n \n return idx;\n } \n};\n```\n\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n- My next post for daily conding challenge\n\nhttps://leetcode.com/problems/minimum-number-of-operations-to-make-array-continuous/solutions/4152964/video-give-me-15-minutes-how-we-think-about-a-solution/\n\n\u2B50\uFE0F Next daily challenge video\nhttps://youtu.be/9DLAvMurzbU\n\n\n- My previous post for daily coding challenge\n\nhttps://leetcode.com/problems/max-dot-product-of-two-subsequences/solutions/4143796/video-give-me-10-minutes-how-we-think-about-a-solution-python-javascript-java-c/\n\n\u2B50\uFE0F Yesterday\'s daily challenge video\n\nhttps://youtu.be/i8QNjFYSHkE | 49 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Python Solution for Finding First and Last Occurrence | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe need to find the first and last occurrence of the given target value in a sorted array of integers.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n1. Check if the target value exists in the input array.\n\n2. If the target value is present in the array:\n \n - `nums.index(target)` \u2192 it finds the first occurrence of the target value in the original array and returns its index\n\n - `nums[::-1].index(target)` \u2192 it finds the first occurrence of the target value in the reversed array and returns its index\n\n - `len(nums) - 1 - ...` \u2192 it calculates the index of the last occurrence of the target value in the original array.\n\n ### Example: \n `nums = [5, 7, 7, 8, 8, 10]`, `target = 8`\n 1. `nums.index(8)` returns `3` because the first 8 is at index 3\n 2. `nums[::-1]` returns `[10, 8, 8, 7, 7, 5]`\n 3. `nums[::-1].index(8)` returns `1` because the first 8 is now at index 1 in the reversed array\n 4. `len(nums) - 1 - ...` length is 6 so, 6 - 1 = 5. Then, we subtract the first occurrence index from 5, which gives us 5 - 1 = `4`\n\n3. Return a list containing both the index of the first and last occurrence of the target value in the original array.\n\n4. If the target is not found in the array, return `[-1, -1]`.\n\n# Complexity\n- Time complexity: $O(n)$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nIn the worst-case scenario, the function may need to examine each element in the list to find the target value. Therefore, the time complexity is O(n), where `n` is the length of the input list. \n\n- Space complexity: $O(1)$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThis is because we are not using any extra data structures that scale with the input size. Regardless of the size of the input list, the function only stores a constant amount of additional information.\n\n# Code\n```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n if target in nums:\n return [nums.index(target), len(nums)-1-nums[::-1].index(target)]\n else:\n return [-1, -1]\n``` | 2 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
✅ Python || Binary Search || Time Complexity O(log(n)) || Beats 98.66% in Python3 || python3 | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | # Intuition\nAs the array is sorted and time complexity is in terms of log(N) , so we can use binary search.\n\n# Approach\n1. Brute Force : we simply Iterate from 0 to n-1 and use flag= True for starting position and storing it, after storing we do flag = False for ending position \nIn this approach Time Complexity is O(N)\n\n2. Binary Search : we use simply Binary Search algorithms , and in return statement (when our conditon get True that is ( nums[mid] == target )) before returing we check for lower index ( that is "index" less than mid ) if their is "lower index" which is less than "mid" index then we return that "index" , and if their is not such "index" means (l_index == -1) so we return "mid" , similarly for right side (ending position ), and we also use "left_bool" which help us in finding starting or ending position (if left_bool = True means we are finding starting position and if left_bool = False means ending position ) \n\n\n# Complexity\n- Time complexity:\nO(log(n))\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n n = len(nums)\n\n res = [-1 , -1]\n\n left = self.binarysearch(nums ,target, 0 , n-1, True )\n if left == -1 :\n return res\n right = self.binarysearch(nums ,target , 0 , n-1, False )\n res[0] = left\n res[1] = right\n\n return res\n \n def binarysearch(self, nums: List[int], t: int , l:int , r:int , left_bool :bool)->int:\n if(l<=r):\n mid = (r+l)//2\n\n if(nums[mid] == t):\n if left_bool :\n l_index = self.binarysearch(nums , t , l , mid-1 ,left_bool )\n if (l_index == -1) :\n return mid \n return l_index\n\n else:\n r_index = self.binarysearch(nums , t , mid+1 , r , left_bool)\n if (r_index == -1):\n return mid \n return r_index\n \n elif(nums[mid] < t):\n return self.binarysearch(nums ,t, mid+1 , r , left_bool)\n else :\n return self.binarysearch(nums ,t, l , mid-1 , left_bool)\n else:\n return -1\n \n\n \n``` | 2 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Python3 Solution: Using Bisect Module | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | ```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n import bisect\n start=bisect.bisect_left(nums,target) \n end=bisect.bisect_right(nums,target)\n if start>end-1:\n return [-1,-1]\n return [start,end-1]\n```\n**Steps:**\n1. Import the \'bisect\' module for efficient binary search operations.\n2. Find the leftmost index where \'target\' could be inserted in \'nums\' while maintaining sorted order.\n3. Find the rightmost index where \'target\' could be inserted in \'nums\' while maintaining sorted order.\n4. If \'start\' is greater than \'end-1\', it means \'target\' is not found in \'nums\'.\n\tReturn [-1, -1] to indicate that the target is not present.\n5. Return the leftmost and rightmost indices of \'target\' in \'nums\'.\n\nReference: https://docs.python.org/3/library/bisect.html \n\n | 1 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Python Solution: Using Bisect Module | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | ```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n import bisect\n start=bisect.bisect_left(nums,target) \n end=bisect.bisect_right(nums,target)\n if start>end-1:\n return [-1,-1]\n return [start,end-1]\n```\n**Steps:**\n1. Import the \'bisect\' module for efficient binary search operations.\n2. Find the leftmost index where \'target\' could be inserted in \'nums\' while maintaining sorted order.\n3. Find the rightmost index where \'target\' could be inserted in \'nums\' while maintaining sorted order.\n4. If \'start\' is greater than \'end-1\', it means \'target\' is not found in \'nums\'.\n\tReturn [-1, -1] to indicate that the target is not present.\n5. Return the leftmost and rightmost indices of \'target\' in \'nums\'.\n\nReference: https://docs.python.org/3/library/bisect.html \n\n | 1 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Easy to Understand || c++ || java || python | find-first-and-last-position-of-element-in-sorted-array | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nWe use a two-pointer approach, where low starts at the beginning of the array, and high starts at the end of the array.\nWe compare the elements at low and high with the target value.\nIf the element at low is less than the target, we increment low.\nIf the element at high is greater than the target, we decrement high.\nIf we find two elements equal to the target, we return their indices in the array as the result.\nIf we do not find any such pair, we return [-1, -1] to indicate that the target value is not present in the array.\n\n\n# Complexity\n- Time complexity:\no(n)\n- Space complexity:\no(1)\n\n# Code\n```\n\n\n```\n```c++ []\nclass Solution {\npublic:\n vector<int> searchRange(vector<int>& nums, int target) {\n vector<int> v;\n int low = 0, high = nums.size() - 1;\n while (low <= high) {\n if (nums[low] < target)\n low = low + 1;\n else if (nums[high] > target)\n high = high - 1;\n else if (nums[low] == target && nums[high] == target) {\n v.push_back(low);\n v.push_back(high);\n return {low, high};\n }\n }\n return {-1, -1};\n }\n};\n\n```\n```python []\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n low, high = 0, len(nums) - 1\n while low <= high:\n if nums[low] < target:\n low += 1\n elif nums[high] > target:\n high -= 1\n elif nums[low] == target and nums[high] == target:\n return [low, high]\n return [-1, -1]\n\n```\n```java []\nclass Solution {\n public int[] searchRange(int[] nums, int target) {\n int[] result = new int[2];\n int low = 0, high = nums.length - 1;\n while (low <= high) {\n if (nums[low] < target) {\n low++;\n } else if (nums[high] > target) {\n high--;\n } else if (nums[low] == target && nums[high] == target) {\n result[0] = low;\n result[1] = high;\n return result;\n }\n }\n result[0] = -1;\n result[1] = -1;\n return result;\n }\n}\n```\n | 7 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Beats : 95.32% [49/145 Top Interview Question] | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | # Intuition\n*O(log n) was hinting, `binary search`.*\n\n# Approach\nThis code block contains two methods: `searchRange` and `binarySearch`. The purpose of this code is to find the range of indices in a sorted list (`nums`) where a given `target` integer appears.\n\nThe `searchRange` method takes two parameters: `nums` (the sorted list of integers) and `target` (the integer we\'re searching for). It returns a list containing two elements - the starting and ending indices of the range where the `target` appears in the list.\n\nHere\'s a step-by-step explanation of how the code works:\n\n1. The `searchRange` method:\n - It initializes two variables `left` and `right` by calling the `binarySearch` method twice. The first call to `binarySearch` with the argument `0` indicates that we\'re searching for the leftmost occurrence of the `target`. The second call with the argument `1` indicates that we\'re searching for the rightmost occurrence of the `target`.\n - The `left` and `right` variables will hold the leftmost and rightmost indices of the `target` in the `nums` list, respectively.\n - Finally, the method returns a list containing the values of `left` and `right`.\n\n2. The `binarySearch` method:\n - This method performs a binary search to find the desired index.\n - It takes three parameters: `nums` (the list), `target` (the value to search for), and `side` (0 for left side, 1 for right side).\n - The method initializes `left` and `right` pointers to the start and end of the list, respectively.\n - It enters a while loop that continues as long as `left` is less than or equal to `right`.\n - In each iteration of the loop, it calculates the middle index (`mid`) of the current segment and checks if the `target` is greater, less, or equal to the element at index `mid`.\n - If `target` is greater, it means the desired value is to the right of `mid`, so it updates `left` to `mid + 1`.\n - If `target` is less, it means the desired value is to the left of `mid`, so it updates `right` to `mid - 1`.\n - If `target` is equal to the element at index `mid`, it updates the `index` variable to `mid` and checks the `side` parameter. If `side` is `0`, it means we\'re searching for the leftmost occurrence, so it moves `right` to `mid - 1`. If `side` is `1`, it means we\'re searching for the rightmost occurrence, so it moves `left` to `mid + 1`.\n - Finally, the method returns the `index` variable, which holds the index of the leftmost or rightmost occurrence of the `target` in the list.\n\n\n\n# Complexity\n- Time complexity:\n O(log n)\n\n- Space complexity:\n O(1)\n\nIn terms of time complexity, each call to the `binarySearch` method performs a `binary search`, which has a time complexity of `O(log n)`, where `n` is the length of the input list `nums`. Since we\'re making two such calls, the overall time complexity of the `searchRange` method is `O(log n)`.\n\nIn terms of `space complexity`, the code uses a constant amount of extra space for variables like `left`, `right`, `index`, and `mid`, so the space complexity is `O(1)`.\n\n# Code\n```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n\n # \'0\' for left side, \'1\' for right side\n left = self.binarySearch(nums, target, 0)\n right = self.binarySearch(nums, target, 1)\n return [left, right]\n\n \n def binarySearch(self, nums, target, side):\n left, right = 0, len(nums) - 1\n index = -1\n while left <= right:\n\n mid = (left + right) // 2\n\n if target > nums[mid]:\n left = mid + 1\n elif target < nums[mid]:\n right = mid - 1\n else:\n index = mid\n if side == 0:\n right = mid - 1\n else:\n left = mid + 1\n return index\n\n``` | 2 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
😎Brute Force to Efficient Method 100% beats🤩 | Java | C++ | Python | JavaScript | C# | PHP | find-first-and-last-position-of-element-in-sorted-array | 1 | 1 | # 1st Method :- Brute force\nThe brute force approach involves iterating through the entire array and checking each element to see if it matches the target element. Here are the steps:\n\n1. Initialize two variables, `first` and `last`, to -1. These variables will be used to store the `first` and last occurrence of the target element.\n2. Iterate through the array using a for loop and check each element:\n - a. If the current element matches the target element and `first` is still -1, set `first` to the current index.\n - b. If the current element matches the target element, update `last` to the current index.\n3. After the loop, return the values of `first` and `last`.\n## Code\n``` Java []\nclass Solution {\n public int[] searchRange(int[] nums, int target) {\n int first = -1; // Initialize first occurrence to -1\n int last = -1; // Initialize last occurrence to -1\n \n // Iterate through the array\n for (int i = 0; i < nums.length; i++) {\n if (nums[i] == target) {\n if (first == -1) {\n first = i; // Found the first occurrence\n }\n last = i; // Update last occurrence\n }\n }\n \n // Return the result as an array\n return new int[]{first, last};\n }\n}\n\n```\n``` C++ []\nclass Solution {\npublic:\n vector<int> searchRange(vector<int>& nums, int target) {\n int first = -1; // Initialize first occurrence to -1\n int last = -1; // Initialize last occurrence to -1\n \n // Iterate through the array\n for (int i = 0; i < nums.size(); i++) {\n if (nums[i] == target) {\n if (first == -1) {\n first = i; // Found the first occurrence\n }\n last = i; // Update last occurrence\n }\n }\n \n // Return the result as a vector\n return {first, last};\n }\n};\n\n```\n``` Python []\nclass Solution:\n def searchRange(self, nums, target):\n first = -1 # Initialize first occurrence to -1\n last = -1 # Initialize last occurrence to -1\n \n # Iterate through the array\n for i in range(len(nums)):\n if nums[i] == target:\n if first == -1:\n first = i # Found the first occurrence\n last = i # Update last occurrence\n \n # Return the result as a list\n return [first, last]\n```\n\n``` JavaScript []\nvar searchRange = function(nums, target) {\n let first = -1; // Initialize first occurrence to -1\n let last = -1; // Initialize last occurrence to -1\n \n // Iterate through the array\n for (let i = 0; i < nums.length; i++) {\n if (nums[i] === target) {\n if (first === -1) {\n first = i; // Found the first occurrence\n }\n last = i; // Update last occurrence\n }\n }\n \n // Return the result as an array\n return [first, last];\n};\n```\n``` C# []\npublic int[] SearchRange(int[] nums, int target) {\n int first = -1; // Initialize first occurrence to -1\n int last = -1; // Initialize last occurrence to -1\n \n // Iterate through the array\n for (int i = 0; i < nums.Length; i++) {\n if (nums[i] == target) {\n if (first == -1) {\n first = i; // Found the first occurrence\n }\n last = i; // Update last occurrence\n }\n }\n \n // Return the result as an array\n return new int[]{first, last};\n}\n```\n``` PHP []\nclass Solution {\n function searchRange($nums, $target) {\n $first = -1; // Initialize first occurrence to -1\n $last = -1; // Initialize last occurrence to -1\n \n $n = count($nums); // Get the length of the array\n \n // Iterate through the array\n for ($i = 0; $i < $n; $i++) {\n if ($nums[$i] == $target) {\n if ($first == -1) {\n $first = $i; // Found the first occurrence\n }\n $last = $i; // Update last occurrence\n }\n }\n \n // Return the result as an array\n return [$first, $last];\n }\n}\n```\n\n\n# Complexity\n#### Time complexity: O(n);\n- The code consists of a single loop that iterates through the entire array of length `n` where `n` is the length of the nums array.\n- Within the loop, it performs constant time operations like comparisons and assignments.\n\nTherefore, the overall time complexity of the code is O(n) because it iterates through the array once.\n#### Space complexity: O(1);\n- The code uses a constant amount of extra space for variables `first` and `last`, which do not depend on the input size.\n- It creates a result array of size 2 to store the `first` and `last` occurrences, which is also constant space.\n\nHence, the space complexity of the code is O(1), indicating that it uses a constant amount of additional space regardless of the input size.\n\n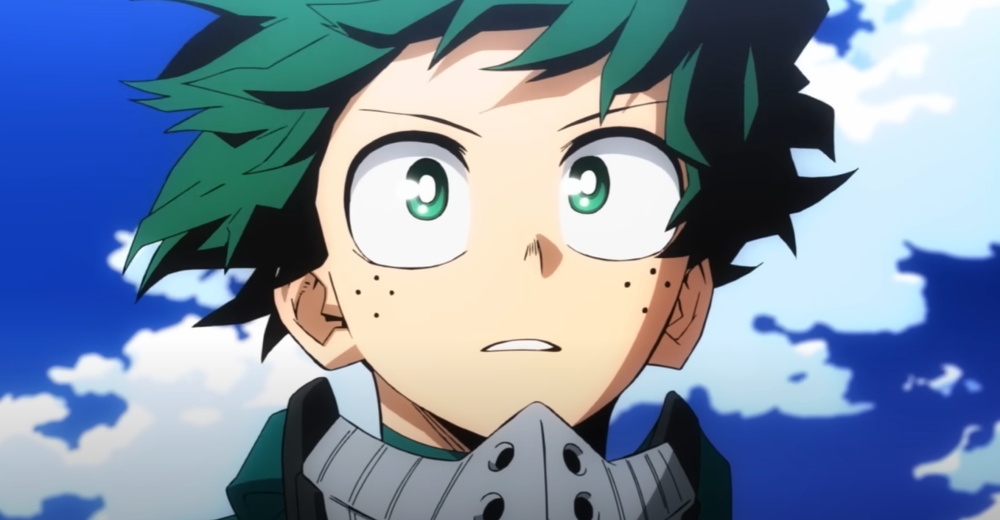\n### plz UpVote\n\n# 2nd Method :- Efficient Method\nNow, let\'s optimize the solution using a more efficient approach. We\'ll use a binary search to find the first and last occurrences of the target element. Here are the steps:\n\n1. Initialize two variables, `first` and `last`, to -1. These variables will be used to store the first and last occurrence of the target element.\n2. Perform a binary search to find the first occurrence:\n - a. Initialize `left` to 0 and `right` to the last index of the array.\n - b. While `left <= right`, calculate the middle index `mid`.\n - c. If `nums[mid]` is equal to the target, update `first` to `mid` and set `right` to `mid - 1` to search in the left half.\n - d. If `nums[mid]` is less than the target, set `left` to `mid + 1`.\n - e. If `nums[mid]` is greater than the target, set `right` to `mid - 1`.\n3. Perform another binary search to find the last occurrence:\n - a. Initialize `left` to 0 and `right` to the last index of the array.\n - b. While `left <= right`, calculate the middle index `mid`.\n - c. If `nums[mid]` is equal to the target, update `last` to `mid` and set `left` to `mid + 1` to search in the right half.\n - d. If `nums[mid]` is less than the target, set `left` to `mid + 1`.\n - e. If `nums[mid]` is greater than the target, set `right` to `mid - 1`.\n4. After both binary searches, return the values of `first` and `last`.\n\n## Code\n``` Java []\nclass Solution {\n public int[] searchRange(int[] nums, int target) {\n int first = findFirst(nums, target); // Find the first occurrence of the target.\n int last = findLast(nums, target); // Find the last occurrence of the target.\n return new int[]{first, last}; // Return the result as an array.\n }\n \n // Helper function to find the first occurrence of the target.\n private int findFirst(int[] nums, int target) {\n int left = 0; // Initialize the left pointer to the beginning of the array.\n int right = nums.length - 1; // Initialize the right pointer to the end of the array.\n int first = -1; // Initialize the variable to store the first occurrence.\n\n while (left <= right) {\n int mid = left + (right - left) / 2; // Calculate the middle index.\n\n if (nums[mid] == target) {\n first = mid; // Update first occurrence.\n right = mid - 1; // Move the right pointer to the left to search in the left half.\n } else if (nums[mid] < target) {\n left = mid + 1; // If mid element is less than target, move the left pointer to the right.\n } else {\n right = mid - 1; // If mid element is greater than target, move the right pointer to the left.\n }\n }\n \n return first; // Return the index of the first occurrence.\n }\n \n // Helper function to find the last occurrence of the target.\n private int findLast(int[] nums, int target) {\n int left = 0; // Initialize the left pointer to the beginning of the array.\n int right = nums.length - 1; // Initialize the right pointer to the end of the array.\n int last = -1; // Initialize the variable to store the last occurrence.\n\n while (left <= right) {\n int mid = left + (right - left) / 2; // Calculate the middle index.\n\n if (nums[mid] == target) {\n last = mid; // Update last occurrence.\n left = mid + 1; // Move the left pointer to the right to search in the right half.\n } else if (nums[mid] < target) {\n left = mid + 1; // If mid element is less than target, move the left pointer to the right.\n } else {\n right = mid - 1; // If mid element is greater than target, move the right pointer to the left.\n }\n }\n \n return last; // Return the index of the last occurrence.\n }\n}\n```\n``` C++ []\nclass Solution {\npublic:\n vector<int> searchRange(vector<int>& nums, int target) {\n int first = findFirst(nums, target); // Find the first occurrence of the target.\n int last = findLast(nums, target); // Find the last occurrence of the target.\n return {first, last}; // Return the result as a vector.\n }\n \n // Helper function to find the first occurrence of the target.\n int findFirst(vector<int>& nums, int target) {\n int left = 0; // Initialize the left pointer to the beginning of the array.\n int right = nums.size() - 1; // Initialize the right pointer to the end of the array.\n int first = -1; // Initialize the variable to store the first occurrence.\n\n while (left <= right) {\n int mid = left + (right - left) / 2; // Calculate the middle index.\n\n if (nums[mid] == target) {\n first = mid; // Update first occurrence.\n right = mid - 1; // Move the right pointer to the left to search in the left half.\n } else if (nums[mid] < target) {\n left = mid + 1; // If mid element is less than target, move the left pointer to the right.\n } else {\n right = mid - 1; // If mid element is greater than target, move the right pointer to the left.\n }\n }\n \n return first; // Return the index of the first occurrence.\n }\n \n // Helper function to find the last occurrence of the target.\n int findLast(vector<int>& nums, int target) {\n int left = 0; // Initialize the left pointer to the beginning of the array.\n int right = nums.size() - 1; // Initialize the right pointer to the end of the array.\n int last = -1; // Initialize the variable to store the last occurrence.\n\n while (left <= right) {\n int mid = left + (right - left) / 2; // Calculate the middle index.\n\n if (nums[mid] == target) {\n last = mid; // Update last occurrence.\n left = mid + 1; // Move the left pointer to the right to search in the right half.\n } else if (nums[mid] < target) {\n left = mid + 1; // If mid element is less than target, move the left pointer to the right.\n } else {\n right = mid - 1; // If mid element is greater than target, move the right pointer to the left.\n }\n }\n \n return last; // Return the index of the last occurrence.\n }\n};\n\n```\n``` Python []\nclass Solution:\n def searchRange(self, nums, target):\n # Helper function to find the first occurrence of the target.\n def findFirst(nums, target):\n left, right = 0, len(nums) - 1\n first = -1\n\n while left <= right:\n mid = left + (right - left) // 2 # Calculate the middle index.\n\n if nums[mid] == target:\n first = mid # Update first occurrence.\n right = mid - 1 # Move the right pointer to the left to search in the left half.\n elif nums[mid] < target:\n left = mid + 1 # If mid element is less than target, move the left pointer to the right.\n else:\n right = mid - 1 # If mid element is greater than target, move the right pointer to the left.\n\n return first\n\n # Helper function to find the last occurrence of the target.\n def findLast(nums, target):\n left, right = 0, len(nums) - 1\n last = -1\n\n while left <= right:\n mid = left + (right - left) // 2 # Calculate the middle index.\n\n if nums[mid] == target:\n last = mid # Update last occurrence.\n left = mid + 1 # Move the left pointer to the right to search in the right half.\n elif nums[mid] < target:\n left = mid + 1 # If mid element is less than target, move the left pointer to the right.\n else:\n right = mid - 1 # If mid element is greater than target, move the right pointer to the left.\n\n return last\n\n # Find the first and last occurrences of the target using the helper functions.\n first = findFirst(nums, target)\n last = findLast(nums, target)\n\n return [first, last] # Return the result as a list.\n\n```\n``` JavaScript []\nvar searchRange = function(nums, target) {\n let first = findFirst(nums, target); // Find the first occurrence of the target.\n let last = findLast(nums, target); // Find the last occurrence of the target.\n return [first, last]; // Return the result as an array.\n};\n\n// Helper function to find the first occurrence of the target.\nfunction findFirst(nums, target) {\n let left = 0; // Initialize the left pointer to the beginning of the array.\n let right = nums.length - 1; // Initialize the right pointer to the end of the array.\n let first = -1; // Initialize the variable to store the first occurrence.\n\n while (left <= right) {\n let mid = left + Math.floor((right - left) / 2); // Calculate the middle index.\n\n if (nums[mid] === target) {\n first = mid; // Update first occurrence.\n right = mid - 1; // Move the right pointer to the left to search in the left half.\n } else if (nums[mid] < target) {\n left = mid + 1; // If mid element is less than target, move the left pointer to the right.\n } else {\n right = mid - 1; // If mid element is greater than target, move the right pointer to the left.\n }\n }\n \n return first; // Return the index of the first occurrence.\n}\n\n// Helper function to find the last occurrence of the target.\nfunction findLast(nums, target) {\n let left = 0; // Initialize the left pointer to the beginning of the array.\n let right = nums.length - 1; // Initialize the right pointer to the end of the array.\n let last = -1; // Initialize the variable to store the last occurrence.\n\n while (left <= right) {\n let mid = left + Math.floor((right - left) / 2); // Calculate the middle index.\n\n if (nums[mid] === target) {\n last = mid; // Update last occurrence.\n left = mid + 1; // Move the left pointer to the right to search in the right half.\n } else if (nums[mid] < target) {\n left = mid + 1; // If mid element is less than target, move the left pointer to the right.\n } else {\n right = mid - 1; // If mid element is greater than target, move the right pointer to the left.\n }\n }\n \n return last; // Return the index of the last occurrence.\n}\n\n```\n``` C# []\npublic int[] SearchRange(int[] nums, int target) {\n int first = FindFirst(nums, target); // Find the first occurrence of the target.\n int last = FindLast(nums, target); // Find the last occurrence of the target.\n return new int[]{first, last}; // Return the result as an array.\n}\n\n// Helper function to find the first occurrence of the target.\nprivate int FindFirst(int[] nums, int target) {\n int left = 0; // Initialize the left pointer to the beginning of the array.\n int right = nums.Length - 1; // Initialize the right pointer to the end of the array.\n int first = -1; // Initialize the variable to store the first occurrence.\n\n while (left <= right) {\n int mid = left + (right - left) / 2; // Calculate the middle index.\n\n if (nums[mid] == target) {\n first = mid; // Update first occurrence.\n right = mid - 1; // Move the right pointer to the left to search in the left half.\n } else if (nums[mid] < target) {\n left = mid + 1; // If mid element is less than target, move the left pointer to the right.\n } else {\n right = mid - 1; // If mid element is greater than target, move the right pointer to the left.\n }\n }\n \n return first; // Return the index of the first occurrence.\n}\n\n// Helper function to find the last occurrence of the target.\nprivate int FindLast(int[] nums, int target) {\n int left = 0; // Initialize the left pointer to the beginning of the array.\n int right = nums.Length - 1; // Initialize the right pointer to the end of the array.\n int last = -1; // Initialize the variable to store the last occurrence.\n\n while (left <= right) {\n int mid = left + (right - left) / 2; // Calculate the middle index.\n\n if (nums[mid] == target) {\n last = mid; // Update last occurrence.\n left = mid + 1; // Move the left pointer to the right to search in the right half.\n } else if (nums[mid] < target) {\n left = mid + 1; // If mid element is less than target, move the left pointer to the right.\n } else {\n right = mid - 1; // If mid element is greater than target, move the right pointer to the left.\n }\n }\n \n return last; // Return the index of the last occurrence.\n}\n\n```\n``` PHP []\nclass Solution {\n function searchRange($nums, $target) {\n $first = $this->findFirst($nums, $target); // Find the first occurrence of the target.\n $last = $this->findLast($nums, $target); // Find the last occurrence of the target.\n return [$first, $last]; // Return the result as an array.\n }\n \n // Helper function to find the first occurrence of the target.\n private function findFirst($nums, $target) {\n $left = 0; // Initialize the left pointer to the beginning of the array.\n $right = count($nums) - 1; // Initialize the right pointer to the end of the array.\n $first = -1; // Initialize the variable to store the first occurrence.\n\n while ($left <= $right) {\n $mid = $left + floor(($right - $left) / 2); // Calculate the middle index.\n\n if ($nums[$mid] == $target) {\n $first = $mid; // Update first occurrence.\n $right = $mid - 1; // Move the right pointer to the left to search in the left half.\n } elseif ($nums[$mid] < $target) {\n $left = $mid + 1; // If mid element is less than target, move the left pointer to the right.\n } else {\n $right = $mid - 1; // If mid element is greater than target, move the right pointer to the left.\n }\n }\n \n return $first; // Return the index of the first occurrence.\n }\n \n // Helper function to find the last occurrence of the target.\n private function findLast($nums, $target) {\n $left = 0; // Initialize the left pointer to the beginning of the array.\n $right = count($nums) - 1; // Initialize the right pointer to the end of the array.\n $last = -1; // Initialize the variable to store the last occurrence.\n\n while ($left <= $right) {\n $mid = $left + floor(($right - $left) / 2); // Calculate the middle index.\n\n if ($nums[$mid] == $target) {\n $last = $mid; // Update last occurrence.\n $left = $mid + 1; // Move the left pointer to the right to search in the right half.\n } elseif ($nums[$mid] < $target) {\n $left = $mid + 1; // If mid element is less than target, move the left pointer to the right.\n } else {\n $right = $mid - 1; // If mid element is greater than target, move the right pointer to the left.\n }\n }\n \n return $last; // Return the index of the last occurrence.\n }\n}\n```\n\n# Complexity\n#### Time complexity: O(log n);\n- The code consists of two binary search functions, findFirst and findLast.\n- Each binary search operates on a sorted array of length n.\n- Binary search has a time complexity of O(log n).\nSince we are performing two separate binary searches, the overall time complexity of the searchRange function is O(log n) for each binary search, resulting in a total time complexity of O(log n) for the entire algorithm.\n\n#### Space complexity: O(1);\n- The space complexity of the code is primarily determined by the space used for variables and function call stack during recursion.\n- The primary variables used are `left`, `right`, `first`, `last`, and `mid`. These variables have a constant space requirement, so they do not contribute significantly to space complexity.\n- There is no significant use of data structures that would consume additional space proportional to the input size.\n\nTherefore, the space complexity of the given code is O(1), which means it uses a constant amount of extra space regardless of the input size. | 15 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
🚩Beats 100% 🔥with 📈easy and optimised approach | 🚀Using Binary Search with Expanding Range | find-first-and-last-position-of-element-in-sorted-array | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe intuition behind this question is to perform two binary searches separately to find the leftmost and rightmost occurrences of the target value.\n\n---\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Implement two binary search functions, one to find the leftmost occurrence and another to find the rightmost occurrence.\n2. Use the binary search to locate the target value in the array.\n3. When the target is found, update the search range for the leftmost occurrence accordingly.\n4. Continue searching for the leftmost occurrence until the left and right pointers converge, and return the leftmost index.\n5. Repeat the binary search for the rightmost occurrence by adjusting the search range accordingly.\n6. Continue searching for the rightmost occurrence until the left and right pointers converge, and return the rightmost index.\n7. If the target value is not found during the binary search, return [-1, -1].\n8. Combine the results from the leftmost and rightmost binary searches and return them as the final result.\n\n---\n\n## Do Upvote if you like the solution and explanation please\n\n---\n\n\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity of this approach is O(log n), where n is the number of elements in the input array. Each binary search operation reduces the search space by half in each step, resulting in logarithmic time complexity.\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity is O(1) because it only uses a constant amount of extra space to store the left, right, and mid pointers and the result vector. The space usage does not depend on the size of the input array.\n\n---\n\n# Code\n```\nclass Solution {\npublic:\n vector<int> searchRange(vector<int>& nums, int target) {\n vector<int> result = {-1, -1};\n int left = binarySearch(nums, target, true);\n if (left == -1) {\n return result;\n }\n int right = binarySearch(nums, target, false);\n result[0] = left;\n result[1] = right;\n return result;\n }\n \n int binarySearch(vector<int>& nums, int target, bool findLeft) {\n int left = 0, right = nums.size() - 1;\n int index = -1;\n \n while (left <= right) {\n int mid = left + (right - left) / 2;\n \n if (nums[mid] == target) {\n index = mid;\n \n if (findLeft) {\n right = mid - 1;\n } else {\n left = mid + 1;\n }\n } else if (nums[mid] < target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n \n return index;\n }\n};\n\n``` | 5 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
C++/Python lower_bound & upper_bound(bisect) vs self made binary search | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nUse C++ lower_bound & upper_bound to solve this question. Python version uses bisect.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n2nd approach is self made binarch search. Use it twice and solve this question. The self made `binary_search` function is similar to C++ lower_bound. \n\nOn the 2nd applying of `binary_search` function, the parameters `nums, target+1` are input. And at final, it needs a check for `nums[j]==target`!\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n\\log n)$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n# Code\n```Python []\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n n=len(nums)\n if n==0: return [-1, -1]\n i=bisect.bisect_left(nums, target)\n if i==n or nums[i]>target: return [-1, -1]\n j=bisect.bisect_right(nums, target)\n return [i, j-1]\n \n```\n```C++ []\n\nclass Solution {\npublic:\n vector<int> searchRange(vector<int>& nums, int target) {\n int n=nums.size();\n if (n==0) return {-1, -1};\n int i=lower_bound(nums.begin(), nums.end(), target)-nums.begin();\n if (i==n || nums[i]>target) return {-1, -1};\n int j=upper_bound(nums.begin(), nums.end(), target)-nums.begin();\n // cout<<i<<","<<j-1<<endl;\n return {i, j-1};\n }\n};\n```\n# Code using custom binary search\n```\nclass Solution {\npublic:\n int n;\n int binary_search(vector<int>& nums, int target){\n int l=0, r=n-1, m;\n while(l<r){\n m=(r+l)/2;\n if (nums[m]<target) l=m+1;\n else r=m;\n }\n return l;\n }\n vector<int> searchRange(vector<int>& nums, int target) {\n n=nums.size();\n if (n==0) return {-1, -1};\n int i=binary_search(nums, target);\n if (nums[i]!=target) return {-1, -1};\n int j=binary_search(nums, target+1);\n if (nums[j]==target) return {i, j}; // j might be n-1. Need this check\n return {i, j-1};\n }\n};\n\n``` | 6 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Simple Solution. Binary search. Python. JavaScript | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n# Binary search for left and right bounds.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Use binary search to find the target\'s index from left side of an array and from the right side of an array.\n# Complexity\n- Time complexity:$$O(n)$$ \n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(1)$$ \n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```javascript []\nvar searchRange = function(nums, target) {\n if (!nums.includes(target)) {\n return [ -1, -1 ]\n }\n const left = left_bound(nums, target);\n const right = right_bound(nums, target);\n\n return [ left, right ]\n};\n\nconst left_bound = (nums, target) => {\n let left = -1;\n let right = nums.length;\n\n while (right - left > 1) {\n let mid = Math.floor((left + right) / 2);\n\n if (nums[mid] < target) {\n left = mid;\n } else {\n right = mid;\n }\n };\n\n return left + 1;\n};\n\nconst right_bound = (nums, target) => {\n let left = -1;\n let right = nums.length;\n\n while (right - left > 1) {\n let mid = Math.floor((left + right) / 2);\n\n if (nums[mid] <= target) {\n left = mid;\n } else {\n right = mid;\n }\n };\n\n return right -1;\n};\n```\n```python []\nclass Solution:\n def left_bound(self, nums, target) -> int:\n left, right = -1, len(nums)\n\n while right - left > 1:\n mid = (right + left) // 2\n if nums[mid] < target:\n left = mid\n else:\n right = mid\n \n return left +1 \n \n def right_bound(self, nums, target) -> int:\n left, right = -1, len(nums)\n\n while right - left > 1:\n mid = (right + left) // 2\n if nums[mid] <= target:\n left = mid\n else:\n right = mid\n \n return right -1\n\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n if target not in nums:\n return [-1, -1]\n left = self.left_bound(nums, target)\n right = self.right_bound(nums, target)\n\n return [left, right]\n```\n\n---\n\n\n# *Please UPVOTE !!!* | 0 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Solution | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nUsing binary search algorithm twice to get the leftmost and rightmost index of the target value.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWriting two binary search in one, one for left and other one for right. \u2014> $$O(2*logn) \u2263 O(logn)$$\n\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(log n)$$\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n\n def bs(arr,target,low,high,left):\n first=-1\n while low<=high:\n mid=(low+high)//2\n if arr[mid]==target:\n first=mid\n if left:\n high=mid-1\n else:\n low=mid+1\n elif arr[mid]>target:\n high=mid-1\n else:low=mid+1\n return first\n\n low=0\n high=len(nums)-1\n return bs(nums,target,low,high,True),bs(nums,target,low,high,False)\n\n``` | 0 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Easy understandable approach Python3 | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | # Intuition\nThe problem asks as to find the range of a given number in a sorted list which is in ascending order like [1,2,4,6,6,6,7] target=6 that means we have return the first occurrence index here it\'s 3 and last occurrence that is 5 something like [3,5] and have to return [-1,-1] if we dont have the given value in the list\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe know that using nums.index(x) returns the index of the first occurrence of x in a list so I used index() two times one to find the starting index and later reversed the array to find the ending index\n\n# Complexity\n- Time complexity:\n The time complexity is O(n) for reversing and using index,I know it doesnt align with the problem but thought it would be nice to know this way also.\nBetween this solution is also accepted\n\n\n\n# Code\n```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n li=[]\n if target in nums:\n # n=c[target]\n li.append(nums.index(target))\n li.append(len(nums)-1-nums[::-1].index(target))\n return li\n \n else:\n return [-1,-1]\n\n``` | 0 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Using Binay search in python.. | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | \nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n l=0\n l1=[]\n h=len(nums)-1\n res1=-1\n res2=-1\n while(l<=h):\n mid=(l+h)//2\n if target==nums[mid]:\n res1=mid\n h=mid-1\n elif target>nums[mid]:\n l=mid+1\n else:\n h=mid-1\n l1.append(res1)\n l=0\n h=len(nums)-1\n while(l<=h):\n mid=(l+h)//2\n if target==nums[mid]:\n res2=mid\n l=mid+1\n elif target>nums[mid]:\n l=mid+1\n else:\n h=mid-1\n l1.append(res2)\n return l1\n``` | 1 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Best solution with tricky method with 99.59% beats || Biggners friendly||line by line explanation | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n```\ndef binarySearch(nums,target):\n```\n\n\ndef binarySearch(nums, target):: This line defines an inner function named binarySearch. It takes two arguments: nums (a list of integers) and target (the value to search for). This function will perform binary search on the given list to find the range of indices where the target value is located.\n\nInside the binarySearch function:\n-----------------------------------\n```\n l=0\n h=len(nums)-1\n while l<=h:\n```\n\nl=0: Initialize a variable l to 0, representing the left boundary of the search range.\nh=len(nums)-1: Initialize a variable h to the index of the last element in nums, representing the right boundary of the search range.\nwhile l<=h:: Start a while loop that continues as long as the left boundary l is less than or equal to the right boundary h. This loop performs binary search within the current search range.\n\nInside the while loop:\n-----------------------\n\n```\n m=(l+h)//2\n \n if nums[m]<target:\n l=m+1\n else:\n h=m-1\n```\n\nm=(l+h)//2: Calculate the middle index m by taking the floor division of the sum of l and h by 2. This is the index where the value of nums will be checked.\n\nif nums[m] < target:: Check if the value at index m in the nums list is less than the target. If it is, it means that the target value must be in the right half of the remaining elements.\n\nUpdate the left boundary l to m + 1 to search in the right half of the remaining elements.\nOtherwise (if nums[m] is greater than or equal to target):\n\nUpdate the right boundary h to m - 1 to search in the left half of the remaining elements.\nreturn [l, h]: When the while loop exits, it means the binary search has completed. Return a list containing the left boundary l and the right boundary h. These boundaries represent the range of indices where the target value would be inserted if it were in the list.\n\nOutside the binarySearch function:\n--------------------------------\n\nCall binarySearch twice:\n-\n```\n left = binarySearch(nums,target-0.1)\n right=binarySearch(nums,target+0.1)\n```\n\n\nleft = binarySearch(nums, target-0.1): Find the range where target - 0.1 would be inserted.\nright = binarySearch(nums, target+0.1): Find the range where target + 0.1 would be inserted.\nCheck if the left boundary of the two ranges is not equal to each other:\n\n\n\n```\n if left[0]!=right[0]:\n return[left[0],right[1]]\n return [-1,-1]\n```\n\nIf they are not equal, it means the target value exists in the nums list.\nIn this case, return [left[0], right[1]], which represents the range of indices where the target value can be found in the list.\nIf the left and right boundaries are equal, it means the target value is not in the list, so return [-1, -1] to indicate that the target value is not present in the list.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(logn)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n def binarySearch(nums,target):\n l=0\n h=len(nums)-1\n while l<=h:\n m=(l+h)//2\n \n if nums[m]<target:\n l=m+1\n else:\n h=m-1\n \n return [l,h]\n left = binarySearch(nums,target-0.1)\n right=binarySearch(nums,target+0.1)\n if left[0]!=right[0]:\n return[left[0],right[1]]\n return [-1,-1]\n \n \n \n \n``` | 1 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
🚩O(n) 📈Simple Solution with detailed explanation | 🚀Using Simple Approach | Beats 100% | find-first-and-last-position-of-element-in-sorted-array | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe intuition behind this question is to count the number of occurrences of the target value in the sorted array and then calculate the starting and ending positions based on the count.\n\n---\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Initialize a count variable to keep track of the number of occurrences of the target value and set it to 0.\n2. Iterate through the array from left to right.\n3. When you encounter the target value, increment the count.\n4. If the count becomes greater than 0, this means you\'ve found at least one occurrence of the target value. Set the starting position to the index of the first occurrence.\n5. Calculate the ending position as the starting position plus the count minus one since all occurrences are adjacent in a sorted array.\n6. Return the starting and ending positions as the result.\n\n---\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity of this approach is O(n), where n is the number of elements in the input array. In the worst case, you may need to traverse the entire array to count the occurrences and find the starting and ending positions.\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity is O(1) because it only uses a constant amount of extra space to store the count and the result vector, regardless of the size of the input array.\n\n---\n\n# Code\n```\nclass Solution {\npublic:\n vector<int> searchRange(vector<int>& nums, int target) {\n vector<int> result = {-1, -1};\n int count = 0;\n \n for (int i = 0; i < nums.size(); ++i) {\n if (nums[i] == target) {\n if (count == 0) {\n result[0] = i;\n }\n count++;\n }\n }\n \n if (count > 0) {\n result[1] = result[0] + count - 1;\n }\n \n return result;\n }\n};\n\n``` | 6 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
Python O(logn) | find-first-and-last-position-of-element-in-sorted-array | 0 | 1 | ```python\nclass Solution:\n def searchRange(self, nums: List[int], target: int) -> List[int]:\n \n def search(x):\n lo, hi = 0, len(nums) \n while lo < hi:\n mid = (lo + hi) // 2\n if nums[mid] < x:\n lo = mid+1\n else:\n hi = mid \n return lo\n \n lo = search(target)\n hi = search(target+1)-1\n \n if lo <= hi:\n return [lo, hi]\n \n return [-1, -1]\n``` | 222 | Given an array of integers `nums` sorted in non-decreasing order, find the starting and ending position of a given `target` value.
If `target` is not found in the array, return `[-1, -1]`.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[5,7,7,8,8,10\], target = 8
**Output:** \[3,4\]
**Example 2:**
**Input:** nums = \[5,7,7,8,8,10\], target = 6
**Output:** \[-1,-1\]
**Example 3:**
**Input:** nums = \[\], target = 0
**Output:** \[-1,-1\]
**Constraints:**
* `0 <= nums.length <= 105`
* `-109 <= nums[i] <= 109`
* `nums` is a non-decreasing array.
* `-109 <= target <= 109` | null |
[VIDEO] Visualization of Binary Search Solution | search-insert-position | 0 | 1 | https://youtu.be/v4J_AWp-6EQ\n\nIf we simply iterated over the entire array to find the target, the algorithm would run in O(n) time. We can improve this by using binary search instead to find the element, which runs in O(log n) time (see the video for a review of binary search).\n\nNow, why does binary search run in O(log n) time? Well, with each iteration, we eliminate around half of the array. So now the question is: how many iterations does it take to converge on a single element? In other words, how many times do we need to divide `n` by 2 until we reach 1?\n\nIf <b>k</b> is the number of times we need to divide `n` by 2 to reach 1, then the equation is:\n\nn / 2<sup>k</sup> = 1\n\nn = 2<sup>k</sup> (multiply both sides by 2<sup>k</sup>)\n\nlog<sub>2</sub>n = k (definition of logarithms)\n\nSo we know that it takes log<sub>2</sub>n steps in the worst case to find the element. But in Big O notation, we drop the base, so this ends up running in O(log n) time.\n\nIf the target was not in the array, then we need to figure out what index the target <i>would</i> be at if it were inserted in sorted order. For a detailed visualization, please see the video (it\'s difficult to describe here) but basically, at the end of the loop, the left pointer will have passed the right pointer (so l > r) and the target needs to be inserted between them. Inserting at index `r` actually ends up inserting the target at 1 spot behind the correct spot, so inserting at index `l` is the correct answer.\n\n# Code\n```\nclass Solution(object):\n def searchInsert(self, nums, target):\n l = 0\n r = len(nums) - 1\n while l <= r:\n mid = (l + r) // 2\n if nums[mid] < target:\n l = mid + 1\n elif nums[mid] > target:\n r = mid - 1\n else:\n return mid\n return l\n \n``` | 7 | Given a sorted array of distinct integers and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[1,3,5,6\], target = 5
**Output:** 2
**Example 2:**
**Input:** nums = \[1,3,5,6\], target = 2
**Output:** 1
**Example 3:**
**Input:** nums = \[1,3,5,6\], target = 7
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 104`
* `-104 <= nums[i] <= 104`
* `nums` contains **distinct** values sorted in **ascending** order.
* `-104 <= target <= 104` | null |
💯 % || Easy Full Explanation || Beginner Friendly🔥🔥 | search-insert-position | 1 | 1 | # Intuition\nSince the array is sorted and we need to achieve O(log n) runtime complexity, we can use binary search. Binary search is an efficient algorithm for finding a target element in a sorted array.\n\n# Approach\n**Initialize Pointers:**\n- Initialize two pointers, low and high, to represent the range of elements in the array where the target may exist. Initially, set low = 0 and high = nums.length - 1.\n\n**Binary Search:**\n- Perform a binary search within the range [low, high].\n- At each step, calculate the middle index as mid = (low + high) / 2.\n- Compare the element at the middle index (nums[mid]) with the target.\n\n**Adjust Pointers:**\n- If nums[mid] is equal to the target, return mid as the index where the target is found.\n- If nums[mid] is less than the target, adjust low to mid + 1.\n- If nums[mid] is greater than the target, adjust high to mid - 1.\n\n**Handle Insertion Position:**\n- If the loop exits without finding the target, return the current value of low as the index where the target would be inserted.\n\n# Complexity\n- **Time complexity:**\nThe time complexity of the provided solution is O(log n). This is because, in each iteration of the binary search, the search space is divided in half. The logarithmic time complexity arises from the fact that the algorithm repeatedly eliminates half of the remaining elements until the target is found or the search space is empty.\n\n- **Space complexity:**\nThe space complexity is O(1). The algorithm uses a constant amount of extra space regardless of the size of the input array. It only uses a few variables (low, high, mid) to perform the search, and the space required for these variables is independent of the input size. Therefore, the space complexity is constant.\n\n```c++ []\nclass Solution {\npublic:\n int searchInsert(vector<int>& nums, int target) {\n int low = 0;\n int high = nums.size() - 1;\n\n while (low <= high) {\n int mid = low + (high - low) / 2;\n\n if (nums[mid] == target) {\n return mid; // Target found\n } else if (nums[mid] < target) {\n low = mid + 1; // Adjust low pointer\n } else {\n high = mid - 1; // Adjust high pointer\n }\n }\n return low; // Return the index for insertion position\n }\n};\n}\n```\n```java []\nclass Solution {\n public int searchInsert(int[] nums, int target) {\n int low = 0;\n int high = nums.length - 1;\n\n while (low <= high) {\n int mid = low + (high - low) / 2;\n\n if (nums[mid] == target) {\n return mid; // Target found\n } else if (nums[mid] < target) {\n low = mid + 1; // Adjust low pointer\n } else {\n high = mid - 1; // Adjust high pointer\n }\n }\n return low; // Return the index for insertion position\n }\n}\n```\n```python []\nclass Solution:\n def search_insert(nums, target):\n low, high = 0, len(nums) - 1\n\n while low <= high:\n mid = (low + high) // 2\n\n if nums[mid] == target:\n return mid # Target found\n elif nums[mid] < target:\n low = mid + 1 # Adjust low pointer\n else:\n high = mid - 1 # Adjust high pointer\n\n return low # Return the index for insertion position\n\n```\n```javascript []\nclass Solution {\n searchInsert(nums, target) {\n let low = 0;\n let high = nums.length - 1;\n\n while (low <= high) {\n let mid = Math.floor((low + high) / 2);\n\n if (nums[mid] === target) {\n return mid; // Target found\n } else if (nums[mid] < target) {\n low = mid + 1; // Adjust low pointer\n } else {\n high = mid - 1; // Adjust high pointer\n }\n }\n\n return low; // Return the index for insertion position\n }\n}\n```\n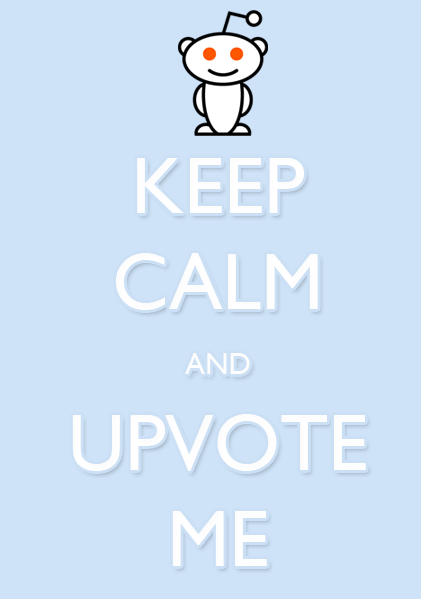\n\n | 13 | Given a sorted array of distinct integers and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[1,3,5,6\], target = 5
**Output:** 2
**Example 2:**
**Input:** nums = \[1,3,5,6\], target = 2
**Output:** 1
**Example 3:**
**Input:** nums = \[1,3,5,6\], target = 7
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 104`
* `-104 <= nums[i] <= 104`
* `nums` contains **distinct** values sorted in **ascending** order.
* `-104 <= target <= 104` | null |
Python Easy Solution || 100% || Binary Search || | search-insert-position | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(logn)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def searchInsert(self, nums: List[int], target: int) -> int:\n l=0\n r=len(nums)-1\n while l<=r: \n mid=(l+r)>>1\n if nums[mid]==target:\n return mid\n elif nums[mid]<target:\n l=mid+1\n else:\n r=mid-1\n if r==mid:\n return mid+1\n else:\n return mid\n``` | 1 | Given a sorted array of distinct integers and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[1,3,5,6\], target = 5
**Output:** 2
**Example 2:**
**Input:** nums = \[1,3,5,6\], target = 2
**Output:** 1
**Example 3:**
**Input:** nums = \[1,3,5,6\], target = 7
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 104`
* `-104 <= nums[i] <= 104`
* `nums` contains **distinct** values sorted in **ascending** order.
* `-104 <= target <= 104` | null |
Easy solution | search-insert-position | 0 | 1 | if the first one doesn\'t work, the second one will work\n\n# Code\n```\nclass Solution:\n def searchInsert(self, nums: List[int], target: int) -> int:\n for i in range(len(nums)):\n if nums[i]==target:\n return i\n nums.append(target)\n nums = sorted(nums) \n for i in range(len(nums)):\n if nums[i]==target:\n return i \n \n \n``` | 0 | Given a sorted array of distinct integers and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[1,3,5,6\], target = 5
**Output:** 2
**Example 2:**
**Input:** nums = \[1,3,5,6\], target = 2
**Output:** 1
**Example 3:**
**Input:** nums = \[1,3,5,6\], target = 7
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 104`
* `-104 <= nums[i] <= 104`
* `nums` contains **distinct** values sorted in **ascending** order.
* `-104 <= target <= 104` | null |
Index Finder in a list | search-insert-position | 0 | 1 | # Intuition\nFirst understand the problem if ur a beginner\n\n# Approach\nI divide these problem into subproblems i spilt it by 3 parts first part is target value is greater than max depends on the i code inside the first part,Second part as target value is present or not if presented then depended program is executed.If ur a beginer then definitly u can understand. \n\n# Complexity\n- Time complexity:\n\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def searchInsert(self, nums: List[int], t: int) -> int:\n n=len(nums)\n mx=max(nums)\n res=0\n if(t>mx):\n res=(n-1)+1\n print(res)\n elif(t in nums):\n for i in range(0,n,1):\n if(nums[i]==t):\n res=i\n else:\n for i in range(0,n-1,1):\n j=i+1\n if(nums[i]<t and nums[j]>t):\n res=j\n\n return res\n\n \n``` | 0 | Given a sorted array of distinct integers and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[1,3,5,6\], target = 5
**Output:** 2
**Example 2:**
**Input:** nums = \[1,3,5,6\], target = 2
**Output:** 1
**Example 3:**
**Input:** nums = \[1,3,5,6\], target = 7
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 104`
* `-104 <= nums[i] <= 104`
* `nums` contains **distinct** values sorted in **ascending** order.
* `-104 <= target <= 104` | null |
Easy python solution | search-insert-position | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def searchInsert(self, nums: List[int], target: int) -> int:\n for i in range(len(nums)):\n if nums[i]==target:\n return i\n elif nums[i]>target:\n return i \n else:\n j=len(nums)\n return j \n``` | 2 | Given a sorted array of distinct integers and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[1,3,5,6\], target = 5
**Output:** 2
**Example 2:**
**Input:** nums = \[1,3,5,6\], target = 2
**Output:** 1
**Example 3:**
**Input:** nums = \[1,3,5,6\], target = 7
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 104`
* `-104 <= nums[i] <= 104`
* `nums` contains **distinct** values sorted in **ascending** order.
* `-104 <= target <= 104` | null |
Solution of search insert position problem | search-insert-position | 0 | 1 | # Approach\nSolved using binary search\n\n# Complexity\n- Time complexity:\n$$O(logn)$$ - as we are using binary search, which runs in O(logn) time.\n\n- Space complexity:\n$$O(1)$$ - as, no extra space is required.\n\n# Code\n```\nclass Solution:\n def searchInsert(self, nums: List[int], target: int) -> int:\n R, L = 0, len(nums)-1\n while R <= L:\n MID = R + (L-R)//2\n if nums[MID] == target:\n return MID\n elif nums[MID] > target:\n L = MID - 1\n else:\n R = MID + 1\n if R > L:\n return R\n \n \n``` | 1 | Given a sorted array of distinct integers and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[1,3,5,6\], target = 5
**Output:** 2
**Example 2:**
**Input:** nums = \[1,3,5,6\], target = 2
**Output:** 1
**Example 3:**
**Input:** nums = \[1,3,5,6\], target = 7
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 104`
* `-104 <= nums[i] <= 104`
* `nums` contains **distinct** values sorted in **ascending** order.
* `-104 <= target <= 104` | null |
Beginner Friendly Solution(96.78% || 73.66%) | search-insert-position | 0 | 1 | **Binary Search Approach**\nRuntime: 43ms beats 96.78%\nMemory: 14.7MB beats 73.66%\n\n# Code\n```\nclass Solution:\n def searchInsert(self, nums: List[int], target: int) -> int:\n high = len(nums) - 1\n low = 0\n while high>=low:\n mid = (low+high)//2\n if nums[mid] == target:\n return mid\n elif nums[mid]>target:\n high = mid -1\n elif nums[mid] < target:\n low = mid + 1\n if nums[mid]>target:\n return mid\n return mid+1\n``` | 1 | Given a sorted array of distinct integers and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.
You must write an algorithm with `O(log n)` runtime complexity.
**Example 1:**
**Input:** nums = \[1,3,5,6\], target = 5
**Output:** 2
**Example 2:**
**Input:** nums = \[1,3,5,6\], target = 2
**Output:** 1
**Example 3:**
**Input:** nums = \[1,3,5,6\], target = 7
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 104`
* `-104 <= nums[i] <= 104`
* `nums` contains **distinct** values sorted in **ascending** order.
* `-104 <= target <= 104` | null |
C++ || Java || Python Solution (7 ms Beats 98.94% of users with C++) | valid-sudoku | 1 | 1 | # Intuition\nThe intuition is to use three matrices (representing rows, columns, and 3x3 subgrids) to keep track of the presence of digits in the Sudoku board. We iterate through each cell in the board and check whether the placement of a digit is valid based on the conditions of Sudoku.\n\n# Approach\n1. Initialize three 2D arrays `m`, `m2`, and `m3` of size 9x9, where `m` represents the presence of digits in rows, `m2` in columns, and `m3` in 3x3 subgrids. Initialize each element to 0.\n2. Iterate through each cell in the Sudoku board.\n - If the cell contains a digit (not \'.\'), convert the character to an integer and subtract 1 to get the index (0 to 8).\n - Calculate the subgrid index `k` based on the current row and column.\n```\nk = i / 3 * 3 + j / 3 \nfor those who need to visualize k > here\n\n0 0 0 | 1 1 1 | 2 2 2\n0 0 0 | 1 1 1 | 2 2 2\n0 0 0 | 1 1 1 | 2 2 2\n--------+---------+---------\n3 3 3 | 4 4 4 | 5 5 5\n3 3 3 | 4 4 4 | 5 5 5\n3 3 3 | 4 4 4 | 5 5 5\n--------+----------+--------\n6 6 6 | 7 7 7 | 8 8 8\n6 6 6 | 7 7 7 | 8 8 8\n6 6 6 | 7 7 7 | 8 8 8 \n```\n - Check if the digit is already present in the corresponding row, column, or subgrid. If yes, return false.\n - Update the presence of the digit in the three matrices.\n3. If the entire board satisfies the Sudoku conditions, return true. Otherwise, return false.\n\n# Complexity\n- Time complexity: $$O(n^2)$$, where n is the size of the Sudoku board (9x9).\n- Space complexity: $$O(n^2)$$, as we use three matrices of size 9x9.\n\n# Code\n```cpp []\nclass Solution {\npublic:\n bool isValidSudoku(vector<vector<char>>& board)\n {\n bool m[9][9] = {0}, m2[9][9] = {0}, m3[9][9] = {0};\n\n for (int i = 0; i < 9; i++)\n for (int j = 0; j < 9; j++)\n if (board[i][j] != \'.\') {\n int num = board[i][j] - \'0\' - 1;\n int k = i / 3 * 3 + j / 3;\n\n if (m[i][num] || m2[j][num] || m3[k][num])\n return false;\n\n m[i][num] = m2[j][num] = m3[k][num] = 1;\n }\n\n return true;\n }\n};\n```\n```java []\npublic class Solution {\n public boolean isValidSudoku(char[][] board) {\n boolean[][] m = new boolean[9][9];\n boolean[][] m2 = new boolean[9][9];\n boolean[][] m3 = new boolean[9][9];\n\n for (int i = 0; i < 9; i++)\n for (int j = 0; j < 9; j++)\n if (board[i][j] != \'.\') {\n int num = board[i][j] - \'0\' - 1;\n int k = i / 3 * 3 + j / 3;\n\n if (m[i][num] || m2[j][num] || m3[k][num])\n return false;\n\n m[i][num] = m2[j][num] = m3[k][num] = true;\n }\n\n return true;\n }\n}\n```\n```python []\nclass Solution:\n def isValidSudoku(self, board: List[List[str]]) -> bool:\n m = [[False] * 9 for _ in range(9)]\n m2 = [[False] * 9 for _ in range(9)]\n m3 = [[False] * 9 for _ in range(9)]\n\n for i in range(9):\n for j in range(9):\n if board[i][j] != \'.\':\n num = int(board[i][j]) - 1\n k = i // 3 * 3 + j // 3\n\n if m[i][num] or m2[j][num] or m3[k][num]:\n return False\n\n m[i][num] = m2[j][num] = m3[k][num] = True\n\n return True\n``` | 1 | Determine if a `9 x 9` Sudoku board is valid. Only the filled cells need to be validated **according to the following rules**:
1. Each row must contain the digits `1-9` without repetition.
2. Each column must contain the digits `1-9` without repetition.
3. Each of the nine `3 x 3` sub-boxes of the grid must contain the digits `1-9` without repetition.
**Note:**
* A Sudoku board (partially filled) could be valid but is not necessarily solvable.
* Only the filled cells need to be validated according to the mentioned rules.
**Example 1:**
**Input:** board =
\[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** true
**Example 2:**
**Input:** board =
\[\[ "8 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** false
**Explanation:** Same as Example 1, except with the **5** in the top left corner being modified to **8**. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit `1-9` or `'.'`. | null |
Valid Sudoku Explanation | valid-sudoku | 0 | 1 | # 2023-11-21 || 3rd\n\n# Code\n```\nclass Solution:\n def isValidSudoku(self, board: List[List[str]]) -> bool:\n for i in range(9):\n for j in range(9):\n if board[i][j] != ".":\n target = board[i][j]\n target_i, target_j = i, j\n else:\n continue\n\n for k in range(9):\n if target_j == k:\n continue\n else:\n if target == board[i][k]:\n print("j")\n return False\n\n for l in range(9):\n if target_i == l:\n continue\n else:\n if target == board[l][j]:\n return False\n\n for n in range(9):\n for m in range(9):\n if n == target_i and m == target_j:\n continue\n\n if n//3 == target_i//3 and m//3 == target_j//3:\n if target == board[n][m]:\n return False\n return True\n``` | 1 | Determine if a `9 x 9` Sudoku board is valid. Only the filled cells need to be validated **according to the following rules**:
1. Each row must contain the digits `1-9` without repetition.
2. Each column must contain the digits `1-9` without repetition.
3. Each of the nine `3 x 3` sub-boxes of the grid must contain the digits `1-9` without repetition.
**Note:**
* A Sudoku board (partially filled) could be valid but is not necessarily solvable.
* Only the filled cells need to be validated according to the mentioned rules.
**Example 1:**
**Input:** board =
\[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** true
**Example 2:**
**Input:** board =
\[\[ "8 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** false
**Explanation:** Same as Example 1, except with the **5** in the top left corner being modified to **8**. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit `1-9` or `'.'`. | null |
3rd | valid-sudoku | 0 | 1 | 3\n\n# Code\n```\nclass Solution:\n def isValidSudoku(self, board: List[List[str]]) -> bool:\n digits = ["1","2","3","4","5","6","7","8","9"]\n a = []\n b = []\n c = []\n for i in range(9):\n for j in range(9):\n num = board[i][j]\n if num in digits:\n if num in a:\n return False\n else:\n a.append(num)\n num = board[j][i]\n if num in digits:\n if num in b:\n return False\n else:\n b.append(num)\n num = board[i//3*3 + j//3][i%3*3 + j%3]\n if num in digits:\n if num in c:\n return False\n else:\n c.append(num)\n a.clear()\n b.clear()\n c.clear()\n \n return True\n \n\n \n\n\n``` | 1 | Determine if a `9 x 9` Sudoku board is valid. Only the filled cells need to be validated **according to the following rules**:
1. Each row must contain the digits `1-9` without repetition.
2. Each column must contain the digits `1-9` without repetition.
3. Each of the nine `3 x 3` sub-boxes of the grid must contain the digits `1-9` without repetition.
**Note:**
* A Sudoku board (partially filled) could be valid but is not necessarily solvable.
* Only the filled cells need to be validated according to the mentioned rules.
**Example 1:**
**Input:** board =
\[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** true
**Example 2:**
**Input:** board =
\[\[ "8 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** false
**Explanation:** Same as Example 1, except with the **5** in the top left corner being modified to **8**. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit `1-9` or `'.'`. | null |
Beats 96.78% || Short 7-line Python solution (with detailed explanation) | valid-sudoku | 0 | 1 | \n1)It initializes an empty list called "res", which will be used to store all the valid elements in the board.\n\n2)It loops through each cell in the board using two nested "for" loops.\nFor each cell, it retrieves the value of the element in that cell and stores it in a variable called "element".\n\n3)If the element is not a dot (\'.\'), which means it\'s a valid number, the method adds three tuples to the "res" list:\n\n- The first tuple contains the row index (i) and the element itself.\n- The second tuple contains the element itself and the column index (j).\n- The third tuple contains the floor division of the row index by 3 (i // 3), the floor division of the column index by 3 (j // 3), and the element itself. This tuple represents the 3x3 sub-grid that the current cell belongs to.\n\n4)After processing all the cells, the method checks if the length of "res" is equal to the length of the set of "res".\n\n```\nclass Solution(object):\n def isValidSudoku(self, board):\n res = []\n for i in range(9):\n for j in range(9):\n element = board[i][j]\n if element != \'.\':\n res += [(i, element), (element, j), (i // 3, j // 3, element)]\n return len(res) == len(set(res))\n\n``` | 196 | Determine if a `9 x 9` Sudoku board is valid. Only the filled cells need to be validated **according to the following rules**:
1. Each row must contain the digits `1-9` without repetition.
2. Each column must contain the digits `1-9` without repetition.
3. Each of the nine `3 x 3` sub-boxes of the grid must contain the digits `1-9` without repetition.
**Note:**
* A Sudoku board (partially filled) could be valid but is not necessarily solvable.
* Only the filled cells need to be validated according to the mentioned rules.
**Example 1:**
**Input:** board =
\[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** true
**Example 2:**
**Input:** board =
\[\[ "8 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** false
**Explanation:** Same as Example 1, except with the **5** in the top left corner being modified to **8**. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit `1-9` or `'.'`. | null |
Detailed solution runs in 100ms | valid-sudoku | 0 | 1 | \n# Code\n```py\nclass Solution:\n def isValidSudoku(self, board: List[List[str]]) -> bool:\n def CheckBox(box,seen):\n if box ==".":\n return True\n if box not in seen:\n seen.append(box)\n return True\n else:\n return False\n\n # Check rows \n for line in board:\n seen = [] \n for box in line:\n if not CheckBox(box, seen):\n return False\n \n # Check collums\n for x in range(len(board)):\n seen = []\n for y in range(len(board)):\n box = board[y][x]\n if not CheckBox(box, seen):\n return False\n\n #Check 3x3s\n #get the other 8 from 3x3 from the top left\n dirs = [[1,0],[2,0],[1,1],[2,1],[0,1],[0,2],[1,2],[2,2]]\n for i in range(0,9,3):\n for j in range(0,9,3):\n seen = []\n seen.append(board[i][j]) # add the staring pos top left\n for d in dirs:\n box = board[i+d[0]][j+d[1]]\n if not CheckBox(box, seen):\n return False\n return True\n \n``` | 1 | Determine if a `9 x 9` Sudoku board is valid. Only the filled cells need to be validated **according to the following rules**:
1. Each row must contain the digits `1-9` without repetition.
2. Each column must contain the digits `1-9` without repetition.
3. Each of the nine `3 x 3` sub-boxes of the grid must contain the digits `1-9` without repetition.
**Note:**
* A Sudoku board (partially filled) could be valid but is not necessarily solvable.
* Only the filled cells need to be validated according to the mentioned rules.
**Example 1:**
**Input:** board =
\[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** true
**Example 2:**
**Input:** board =
\[\[ "8 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** false
**Explanation:** Same as Example 1, except with the **5** in the top left corner being modified to **8**. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit `1-9` or `'.'`. | null |
Beats : 96.06% [50/145 Top Interview Question] | valid-sudoku | 0 | 1 | # Intuition\n*A simple straight-forward solution!*\n\n# Approach\nThis code block is an implementation of the solution to `isValidSudoku`. Let\'s break down the code step by step:\n\n1. The code starts by importing the `collections` module, which is used to create defaultdicts (Why? Will explain!).\n \n2. Within the `isValidSudoku` method, three defaultdicts are created: `rows`, `columns`, and `sub_boxes`. These defaultdicts will store sets of numbers that are present in each row, column, and sub-box of the Sudoku board, respectively.\n\n3. The code then uses two nested loops to iterate through each cell in the 9x9 Sudoku board.\n\n4. For each cell, it checks the value of the number in the cell (`num`):\n\n - If the cell contains a period (`.`), which represents an empty cell, it skips the current iteration using `continue`.\n\n - If the cell contains a number, it checks three conditions to determine if the number violates the rules of Sudoku:\n\n - It checks if the number is already present in the set of numbers in the same row (`rows[row]`).\n \n - It checks if the number is already present in the set of numbers in the same column (`columns[col]`).\n \n - It checks if the number is already present in the set of numbers in the corresponding sub-box (`sub_boxes[(row//3, col//3)]`).\n\n - If any of these conditions are met, it means the Sudoku rules are violated, and the function immediately returns `False`, indicating that the Sudoku board is not valid.\n\n5. If none of the conditions are met, meaning the number can be safely placed in the current cell without violating any rules, the code updates the sets in `rows`, `columns`, and `sub_boxes` to include the new number.\n\n6. After iterating through the entire board, if no violations were found, the function returns `True`, indicating that the Sudoku board is valid.\n\n\n# Complexity\n- Time complexity:\n O(1) , ***Actually O(81) since constant\'s are O(1)***\n\n- Space complexity:\n O(1), ***Confusing? It\'s simple. The total space incurred will be three times the size of the Sudoku board, and it\'s constant in length! It might get smaller but will never exceed 3(9+1 x 9+1).***\n\n# Code\n```\nfrom collections import defaultdict\nclass Solution:\n def isValidSudoku(self, board: List[List[str]]) -> bool:\n # Create dictionaries to keep track of numbers in rows, columns, and sub-boxes\n rows = collections.defaultdict(set)\n columns = collections.defaultdict(set)\n sub_boxes = collections.defaultdict(set)\n\n # Iterate through each cell in the 9x9 Sudoku board\n for row in range(9):\n for col in range(9):\n num = board[row][col]\n\n # Skip empty cells represented by "."\n if num == ".":\n continue\n\n # Check if the current number violates Sudoku rules\n if (num in rows[row] or \n num in columns[col] or \n num in sub_boxes[(row // 3, col // 3)]):\n return False\n\n # Update sets to keep track of encountered numbers\n rows[row].add(num)\n columns[col].add(num)\n sub_boxes[(row // 3, col // 3)].add(num)\n\n # If all cells satisfy Sudoku rules, the board is valid\n return True\n\n```\n\n***Extra note:***\n\n*Why we used **defaultdict?**.*\n-- *It is to simplify the process of initializing and accessing values in a dictionary, especially when dealing with keys that might not exist yet. It provides a convenient way to handle default values for keys that are not present in the dictionary.*\n\n\n- `num in sub_boxes[(row // 3, col // 3)]`:\n\n*This line checks whether the number `num` is already present in the set that corresponds to the specific ***3x3*** sub-box where the current cell is located.*\n\n*Imagine you\'re playing a game on a ***9x9*** Sudoku board. To make sure you\'re not violating the rules, you want to know if the number you\'re considering ***(let\'s say "5")*** is already in the little ***3x3*** box that your current cell is part of. You\'d look at that ***3x3*** box, see if the number ***"5"*** is already there, and if it is, you\'d know you can\'t place another ***"5"*** there. This line of code is doing that exact check for you, but programmatically.*\n\n```\n 0 1 2\n - - - | - - - | - - -\n0 - - - | - - - | - - - // sub_boxes key\'s as a tuple (x,y)\n - - - | - - - | - - -\n -----------------------\n - - - | - - - | - - -\n1 - - - | - - - | - - -\n - - - | - - - | - - -\n -----------------------\n - - - | - - - | - - -\n2 - - - | - - - | - - -\n - - - | - - - | - - -\n```\n\n- `sub_boxes[(row // 3, col // 3)].add(num)`:\n\n*This line adds the current number `num` to the set that corresponds to the ***3x3*** sub-box where the current cell is located.*\n\n*Continuing from the previous analogy, let\'s say you found that the number **"5"** isn\'t in the ***3x3*** box yet, so you want to add it there. You take your pen and add the ***"5"*** to the list of numbers you\'ve placed in that box. This line of code does the same thing in code. It adds the current number `num` to the set that represents the numbers placed in that specific ***3x3*** sub-box.*\n\n***In both cases, these lines help ensure that you\'re following the rules of Sudoku by keeping track of the numbers in rows, columns, and sub-boxes, making sure no number repeats within the same row, column, or sub-box.***\n\n***END***\n\n\n*It took a lot of time and effort to write this documentation, but I will be really happy if it becomes useful to someone!* \n\n\n\n | 15 | Determine if a `9 x 9` Sudoku board is valid. Only the filled cells need to be validated **according to the following rules**:
1. Each row must contain the digits `1-9` without repetition.
2. Each column must contain the digits `1-9` without repetition.
3. Each of the nine `3 x 3` sub-boxes of the grid must contain the digits `1-9` without repetition.
**Note:**
* A Sudoku board (partially filled) could be valid but is not necessarily solvable.
* Only the filled cells need to be validated according to the mentioned rules.
**Example 1:**
**Input:** board =
\[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** true
**Example 2:**
**Input:** board =
\[\[ "8 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** false
**Explanation:** Same as Example 1, except with the **5** in the top left corner being modified to **8**. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit `1-9` or `'.'`. | null |
Python | 2 WAYS | Sets / Strings | O(1) Time / Space | 90% T 88% S | valid-sudoku | 0 | 1 | # Solved 2 ways - Utilizing 3 Sets and Building Strings\n\n**Approach 1: Utilizing 3 Sets with HashMaps**\n\nWe want to traverse the sudoku board whilst populating and checking against a suite of 3 sets that may invalidate our board. We know that duplicate elements invalidate the board if they fall within the same row, column, or "sub-box" so we will construct our sets accordingly.\n\n* We first initialize 3 hash tables pointing to sets to store "seen" squares:\n\t* ```Row Set```\n\t* ```Column Set```\n\t* ```Sub-Box Set```\n* We then loop through each sudoku square on our board and check against each of our sets\n* If we find our value in any of our sets, we are safe to return ```False``` as long as we index our hashmaps correctly\n\n**Indexing**\n* ```Row Set Map``` is x-indexed\n* ```Column Set Map``` is y-indexed\n* ```Sub-Box Set Map``` is indexed by tuple ```(x // 3, y // 3)```\n\t* This ensures each coodinate falls into one of the 9 "sub-boxes" within the board\n\n**Complexity**\nTime complexity: O(9 x 9) ->O(1)\nSpace complexity: O(9 x 9 x 3) -> O(1)\n<br>\n\n```\nfrom collections import defaultdict\nclass Solution:\n def isValidSudoku(self, board: List[List[str]]) -> bool:\n \n rows = collections.defaultdict(set)\n cols = collections.defaultdict(set)\n boxes = collections.defaultdict(set)\n \n for x in range(9):\n for y in range(9):\n \n # grab sudoku square \n val = board[x][y]\n \n # only process if it\'s a number\n if val != \'.\':\n \n # check sudoku square against each set\n if val in rows[x] or val in cols[y] or val in boxes[(x // 3, y // 3)]:\n return False\n\n # populate sets with sudoku square\n rows[x].add(val)\n cols[y].add(val)\n # (x // 3, y // 3) "buckets" each board coordinate into corresponding sub-box \n boxes[(x // 3, y // 3)].add(val) \n \n return True\n```\n\n<br>\n\n**Approach 2: Generating Strings and Hashing**\n\nAnother approach is by generating strings of each possible combination that may invalidate our board. In our case, that is each row, column, and sub-box within our board. \n\n* We first loop through each row in our board and generate a corresponding ```rowString```:\n* We then hash each character in our ```rowString``` to its frequency and scan for any duplicates\n* If we find a number with ```frequency > 1``` we can safely return ```False```\n* Repeat these steps for ```colString``` and ```boxString```\n\n**Complexity**\nTime complexity: O(9 x 9) -> O(1)\nSpace complexity: O(9 x 9 x 3) -> O(1)\n<br>\n\n```\nclass Solution:\n def isValidSudoku(self, board: List[List[str]]) -> bool:\n \n # handle rows\n for row in board:\n # generates string / map for each row\n rowString = "".join(row)\n rowCounts = Counter(rowString)\n \n # scans map for duplicate nums\n for key, val in rowCounts.items():\n if key != \'.\' and val > 1:\n return False\n \n # handle cols\n cols = [[] for _ in range(9)]\n \n for r, row in enumerate(board):\n for c, val in enumerate(row):\n cols[c].append(val)\n \n for col in cols:\n # generates string / map for each col\n colString = "".join(col)\n colCounts = Counter(colString)\n \n # scans map for duplicate nums\n for key, val in colCounts.items():\n if key != \'.\' and val > 1:\n return False\n \n # handle sub-boxes\n startingIndices = [[0,0], [0,3], [0,6], [3,0], [3,3], [3,6], [6,0], [6,3], [6,6]]\n \n for x, y in startingIndices:\n # generates string / map for each sub-box\n boxString = ""\n for i in range(3):\n boxString += "".join(board[x + i][y:y+3])\n \n\t\t\tboxCounts = Counter(boxString)\n\t\t\t\n # scans map for duplicate nums\n for key, val in boxCounts.items():\n if key != \'.\' and val > 1:\n return False\n \n return True\n``` | 1 | Determine if a `9 x 9` Sudoku board is valid. Only the filled cells need to be validated **according to the following rules**:
1. Each row must contain the digits `1-9` without repetition.
2. Each column must contain the digits `1-9` without repetition.
3. Each of the nine `3 x 3` sub-boxes of the grid must contain the digits `1-9` without repetition.
**Note:**
* A Sudoku board (partially filled) could be valid but is not necessarily solvable.
* Only the filled cells need to be validated according to the mentioned rules.
**Example 1:**
**Input:** board =
\[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** true
**Example 2:**
**Input:** board =
\[\[ "8 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** false
**Explanation:** Same as Example 1, except with the **5** in the top left corner being modified to **8**. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit `1-9` or `'.'`. | null |
Sudoku Validator: The Art of Efficient Board Inspection in Python | valid-sudoku | 0 | 1 | # Intuition\nThe intuition behind the solution is to iterate through each row, column, and 3x3 box of the Sudoku board, keeping track of the numbers encountered. If a number is repeated in any row, column, or box, the board is not valid.\n# Approach\nThe approach involves using three lists (`row`, `column`, and `box`) to keep track of numbers in each respective context. For each cell in the Sudoku board, check if the number is already in the corresponding list. If yes, the board is invalid. Otherwise, add the number to the list. Repeat this process for all rows, columns, and 3x3 boxes. \n# Complexity\n- Time complexity:\nO(1) since the size of the Sudoku board is fixed (9x9). The double loop iterates through each cell, making it O(1).\n- Space complexity:\nO(1) since the size of the lists (`row`, `column`, and `box`) is constant regardless of the input size.\n# Code\n```\nclass Solution:\n def isValidSudoku(self, board: List[List[str]]) -> bool:\n for i in range(9):\n row = []\n column = []\n box = []\n\n for j in range(9):\n # Check each row\n if board[i][j] != ".":\n if board[i][j] in row:\n return False\n row.append(board[i][j])\n\n # Check each column\n if board[j][i] != ".":\n if board[j][i] in column:\n return False\n column.append(board[j][i])\n\n # Check each 3x3 box\n box_row, box_col = 3 * (i // 3), 3 * (i % 3)\n if board[box_row + j // 3][box_col + j % 3] != ".":\n if board[box_row + j // 3][box_col + j % 3] in box:\n return False\n box.append(board[box_row + j // 3][box_col + j % 3])\n\n return True\n\n``` | 1 | Determine if a `9 x 9` Sudoku board is valid. Only the filled cells need to be validated **according to the following rules**:
1. Each row must contain the digits `1-9` without repetition.
2. Each column must contain the digits `1-9` without repetition.
3. Each of the nine `3 x 3` sub-boxes of the grid must contain the digits `1-9` without repetition.
**Note:**
* A Sudoku board (partially filled) could be valid but is not necessarily solvable.
* Only the filled cells need to be validated according to the mentioned rules.
**Example 1:**
**Input:** board =
\[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** true
**Example 2:**
**Input:** board =
\[\[ "8 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** false
**Explanation:** Same as Example 1, except with the **5** in the top left corner being modified to **8**. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit `1-9` or `'.'`. | null |
[Python] - Clean & Simple - Faster than 96% in runtime 🔥🔥🔥 | valid-sudoku | 0 | 1 | To solve this problem we have to check if each value in sudoku does not repeat in its:\n\n1. Row\n1. Column\n1. Block\n\n# Complexity\n- Time complexity: $$O(n*n)$$ \n where n is fiexed here.\n so, Time Complexity will be O(81) ==> O(1)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n * n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isValidSudoku(self, board: List[List[str]]) -> bool:\n rows = [set() for _ in range(9)]\n cols = [set() for _ in range(9)]\n block = [[set() for _ in range(3)] for _ in range(3)]\n\n for i in range(9):\n for j in range(9):\n curr = board[i][j]\n if curr == \'.\':\n continue\n if (curr in rows[i]) or (curr in cols[j]) or (curr in block[i // 3][j // 3]):\n return False\n rows[i].add(curr)\n cols[j].add(curr)\n block[i // 3][j // 3].add(curr)\n return True\n\n``` | 23 | Determine if a `9 x 9` Sudoku board is valid. Only the filled cells need to be validated **according to the following rules**:
1. Each row must contain the digits `1-9` without repetition.
2. Each column must contain the digits `1-9` without repetition.
3. Each of the nine `3 x 3` sub-boxes of the grid must contain the digits `1-9` without repetition.
**Note:**
* A Sudoku board (partially filled) could be valid but is not necessarily solvable.
* Only the filled cells need to be validated according to the mentioned rules.
**Example 1:**
**Input:** board =
\[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** true
**Example 2:**
**Input:** board =
\[\[ "8 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** false
**Explanation:** Same as Example 1, except with the **5** in the top left corner being modified to **8**. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit `1-9` or `'.'`. | null |
[Python / C++] a simple-minded brute-force solution in Python & CPP | valid-sudoku | 0 | 1 | ```cpp\nclass Solution {\npublic:\n bool isValidSudoku(vector<vector<char>>& board)\n {\n int x, y;\n\n x = -1;\n while (++x < 9)\n {\n y = -1;\n while (++y < 9)\n {\n if (board[x][y] != \'.\' && (!check_row(board, x, y) \n || !check_col(board, x, y) || !check_box(board, x, y)))\n return (false);\n }\n }\n return (true);\n }\n\n /* ------------------------------ */\n\n bool check_row(vector<vector<char>>& board, int x, int y)\n {\n int i = -1;\n \n while (++i < 9)\n {\n if (i != x && board[x][y] == board[i][y])\n return (false);\n }\n return (true);\n }\n \n bool check_col(vector<vector<char>>& board, int x, int y)\n {\n int i = -1;\n \n while (++i < 9)\n {\n if (i != y && board[x][y] == board[x][i])\n return (false);\n }\n return (true);\n }\n \n bool check_box(vector<vector<char>> & board, int x, int y)\n {\n int rr, cc, i, j;\n\n rr = x / 3 * 3;\n cc = y / 3 * 3;\n i = rr - 1;\n while (++i < rr + 3)\n {\n j = cc - 1;\n while (++j < cc + 3)\n {\n if (board[i][j] == board[x][y] && x != i && y != j)\n return (false);\n }\n }\n return (true);\n }\n};\n```\n\n```py\nclass Solution:\n def isValidSudoku(self, board: List[List[str]]) -> bool:\n for r in board:\n d = []\n for c in r:\n if c.isdigit():\n if c in d:\n # print(c,d)\n # print(3)\n return False\n d.append(c)\n for c in range(9):\n d = []\n for r in range(9):\n n = board[r][c]\n if n.isdigit():\n if n in d:\n # print(2)\n return False\n d.append(n)\n for r in range(3):\n for c in range(3):\n d = []\n for rr in range(r * 3, r * 3 + 3):\n for cc in range(c * 3, c * 3 + 3):\n n = board[rr][cc]\n if n.isdigit():\n if n in d:\n # print(1)\n return False\n d.append(n)\n return True\n"""\n[\n[".",".",".",".",".",".","5",".","."],\n[".",".",".",".",".",".",".",".","."],\n[".",".",".",".",".",".",".",".","."],\n["9","3",".",".","2",".","4",".","."],\n[".",".","7",".",".",".","3",".","."],\n[".",".",".",".",".",".",".",".","."],\n[".",".",".","3","4",".",".",".","."],\n[".",".",".",".",".","3",".",".","."],\n[".",".",".",".",".","5","2",".","."]]\n\n[\n[".",".",".",".","5",".",".","1","."],\n[".","4",".","3",".",".",".",".","."],\n[".",".",".",".",".","3",".",".","1"],\n["8",".",".",".",".",".",".","2","."],\n[".",".","2",".","7",".",".",".","."],\n[".","1","5",".",".",".",".",".","."],\n[".",".",".",".",".","2",".",".","."],\n[".","2",".","9",".",".",".",".","."],\n[".",".","4",".",".",".",".",".","."]]\n\n[\n[".",".","4",".",".",".","6","3","."],\n[".",".",".",".",".",".",".",".","."],\n["5",".",".",".",".",".",".","9","."],\n[".",".",".","5","6",".",".",".","."],\n["4",".","3",".",".",".",".",".","1"],\n[".",".",".","7",".",".",".",".","."],\n[".",".",".","5",".",".",".",".","."],\n[".",".",".",".",".",".",".",".","."],\n[".",".",".",".",".",".",".",".","."]]\n\n[\n["5","3",".",".","7",".",".",".","."],\n["6",".",".","1","9","5",".",".","."],\n[".","9","8",".",".",".",".","6","."],\n["8",".",".",".","6",".",".",".","3"],\n["4",".",".","8",".","3",".",".","1"],\n["7",".",".",".","2",".",".",".","6"],\n[".","6",".",".",".",".","2","8","."],\n[".",".",".","4","1","9",".",".","5"],\n[".",".",".",".","8",".",".","7","9"]]\n\n[[".","8","7","6","5","4","3","2","1"],\n["2",".",".",".",".",".",".",".","."],\n["3",".",".",".",".",".",".",".","."],\n["4",".",".",".",".",".",".",".","."],\n["5",".",".",".",".",".",".",".","."],\n["6",".",".",".",".",".",".",".","."],\n["7",".",".",".",".",".",".",".","."],\n["8",".",".",".",".",".",".",".","."],\n["9",".",".",".",".",".",".",".","."]]\n"""\n``` | 3 | Determine if a `9 x 9` Sudoku board is valid. Only the filled cells need to be validated **according to the following rules**:
1. Each row must contain the digits `1-9` without repetition.
2. Each column must contain the digits `1-9` without repetition.
3. Each of the nine `3 x 3` sub-boxes of the grid must contain the digits `1-9` without repetition.
**Note:**
* A Sudoku board (partially filled) could be valid but is not necessarily solvable.
* Only the filled cells need to be validated according to the mentioned rules.
**Example 1:**
**Input:** board =
\[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** true
**Example 2:**
**Input:** board =
\[\[ "8 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** false
**Explanation:** Same as Example 1, except with the **5** in the top left corner being modified to **8**. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit `1-9` or `'.'`. | null |
Python solutions | Single Traversal | Single Dictionary | valid-sudoku | 0 | 1 | Just store the indexs of the numbers in a dictionary in `(x, y)` format. Then for every number check for same row, same col and same box condition.\nThis will require a single traversal. The same box condition can be checked using `pos[0]//3 == x//3 and pos[1]//3 == y//3` since `i//3` and `j//3` give the box position.\n\n```\nclass Solution:\n def isValidSudoku(self, board: List[List[str]]) -> bool:\n boardMap = collections.defaultdict(list)\n for x in range(9):\n for y in range(9):\n char = board[x][y]\n if char != \'.\': \n if char in boardMap:\n for pos in boardMap[char]:\n if (pos[0]== x) or (pos[1] == y) or (pos[0]//3 == x//3 and pos[1]//3 == y//3):\n return False\n boardMap[char].append((x,y))\n \n return True\n``` | 78 | Determine if a `9 x 9` Sudoku board is valid. Only the filled cells need to be validated **according to the following rules**:
1. Each row must contain the digits `1-9` without repetition.
2. Each column must contain the digits `1-9` without repetition.
3. Each of the nine `3 x 3` sub-boxes of the grid must contain the digits `1-9` without repetition.
**Note:**
* A Sudoku board (partially filled) could be valid but is not necessarily solvable.
* Only the filled cells need to be validated according to the mentioned rules.
**Example 1:**
**Input:** board =
\[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** true
**Example 2:**
**Input:** board =
\[\[ "8 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\]
,\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\]
,\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\]
,\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\]
,\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\]
,\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\]
,\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\]
,\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\]
,\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** false
**Explanation:** Same as Example 1, except with the **5** in the top left corner being modified to **8**. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit `1-9` or `'.'`. | null |
Easy Solution | sudoku-solver | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom typing import List\n\nclass Solution:\n def solveSudoku(self, board: List[List[str]]) -> None:\n """\n Solves the Sudoku puzzle in-place.\n\n Parameters:\n board (List[List[str]]): 9x9 Sudoku board represented as a list of lists.\n """\n n = 9\n\n def isValid(row, col, num):\n for i in range(n):\n if board[i][col] == num or board[row][i] == num or board[3 * (row // 3) + i // 3][3 * (col // 3) + i % 3] == num:\n return False\n return True\n\n def solve(row, col):\n if row == n:\n return True\n if col == n:\n return solve(row + 1, 0)\n\n if board[row][col] == ".":\n for num in map(str, range(1, 10)):\n if isValid(row, col, num):\n board[row][col] = num\n if solve(row, col + 1):\n return True\n else:\n board[row][col] = "."\n return False\n else:\n return solve(row, col + 1)\n\n solve(0, 0)\n##Upvote me If it helps please\n\n``` | 6 | Write a program to solve a Sudoku puzzle by filling the empty cells.
A sudoku solution must satisfy **all of the following rules**:
1. Each of the digits `1-9` must occur exactly once in each row.
2. Each of the digits `1-9` must occur exactly once in each column.
3. Each of the digits `1-9` must occur exactly once in each of the 9 `3x3` sub-boxes of the grid.
The `'.'` character indicates empty cells.
**Example 1:**
**Input:** board = \[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\],\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\],\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\],\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\],\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\],\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\],\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\],\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\],\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** \[\[ "5 ", "3 ", "4 ", "6 ", "7 ", "8 ", "9 ", "1 ", "2 "\],\[ "6 ", "7 ", "2 ", "1 ", "9 ", "5 ", "3 ", "4 ", "8 "\],\[ "1 ", "9 ", "8 ", "3 ", "4 ", "2 ", "5 ", "6 ", "7 "\],\[ "8 ", "5 ", "9 ", "7 ", "6 ", "1 ", "4 ", "2 ", "3 "\],\[ "4 ", "2 ", "6 ", "8 ", "5 ", "3 ", "7 ", "9 ", "1 "\],\[ "7 ", "1 ", "3 ", "9 ", "2 ", "4 ", "8 ", "5 ", "6 "\],\[ "9 ", "6 ", "1 ", "5 ", "3 ", "7 ", "2 ", "8 ", "4 "\],\[ "2 ", "8 ", "7 ", "4 ", "1 ", "9 ", "6 ", "3 ", "5 "\],\[ "3 ", "4 ", "5 ", "2 ", "8 ", "6 ", "1 ", "7 ", "9 "\]\]
**Explanation:** The input board is shown above and the only valid solution is shown below:
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit or `'.'`.
* It is **guaranteed** that the input board has only one solution. | null |
Simple Backtracking Approach | sudoku-solver | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe Sudoku puzzle can be solved using a backtracking algorithm. We\'ll start by iterating through the entire board, looking for empty cells. For each empty cell, we\'ll try to fill it with numbers from \'1\' to \'9\' while ensuring that the Sudoku rules are satisfied. If we reach an invalid state, we\'ll backtrack and try a different number until a valid solution is found or it\'s determined that the puzzle is unsolvable.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe Create a fuction `def isValid` to check if we can place the number `k` at the position r,c passed as a parameter to the isValid function.\n\nIn the isValid function we check if k is already present in the same row r or the column c by iterating from 0-8. In the same iteration we find if k is already present in that block of puzzle by dividing the puzzle in 9 blocks of 3x3 size. If all of these condition fails then we return True else False.\n\nIf no value between 1-9 can be placed then we backtrack and change the value of the earlier placed position. \n\n\n# Code\n```\nclass Solution:\n def solveSudoku(self, board: List[List[str]]) -> None:\n def isValid(r,c,k):\n for i in range(0,9): \n if board[i][c]==k:\n print(board[i][c],k) \n return False\n if board[r][i]==k: \n return False\n if board[3*(r//3) + i//3][3*(c//3) + i%3]==k: \n return False\n return True \n\n def fill(r,c):\n if r==9:\n return True\n if c==9:\n return fill(r+1,0) \n if board[r][c]==\'.\':\n for k in range(1,10):\n if isValid(r,c,str(k))==True:\n board[r][c]=str(k)\n if fill(r,c+1):\n return True\n board[r][c]=\'.\'\n return False\n return fill(r,c+1) \n\n fill(0,0)\n\n #Upvote if it helps\n``` | 4 | Write a program to solve a Sudoku puzzle by filling the empty cells.
A sudoku solution must satisfy **all of the following rules**:
1. Each of the digits `1-9` must occur exactly once in each row.
2. Each of the digits `1-9` must occur exactly once in each column.
3. Each of the digits `1-9` must occur exactly once in each of the 9 `3x3` sub-boxes of the grid.
The `'.'` character indicates empty cells.
**Example 1:**
**Input:** board = \[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\],\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\],\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\],\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\],\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\],\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\],\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\],\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\],\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** \[\[ "5 ", "3 ", "4 ", "6 ", "7 ", "8 ", "9 ", "1 ", "2 "\],\[ "6 ", "7 ", "2 ", "1 ", "9 ", "5 ", "3 ", "4 ", "8 "\],\[ "1 ", "9 ", "8 ", "3 ", "4 ", "2 ", "5 ", "6 ", "7 "\],\[ "8 ", "5 ", "9 ", "7 ", "6 ", "1 ", "4 ", "2 ", "3 "\],\[ "4 ", "2 ", "6 ", "8 ", "5 ", "3 ", "7 ", "9 ", "1 "\],\[ "7 ", "1 ", "3 ", "9 ", "2 ", "4 ", "8 ", "5 ", "6 "\],\[ "9 ", "6 ", "1 ", "5 ", "3 ", "7 ", "2 ", "8 ", "4 "\],\[ "2 ", "8 ", "7 ", "4 ", "1 ", "9 ", "6 ", "3 ", "5 "\],\[ "3 ", "4 ", "5 ", "2 ", "8 ", "6 ", "1 ", "7 ", "9 "\]\]
**Explanation:** The input board is shown above and the only valid solution is shown below:
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit or `'.'`.
* It is **guaranteed** that the input board has only one solution. | null |
Python Easiest Recursive Solution. | sudoku-solver | 0 | 1 | ```\nclass Solution:\n def solveSudoku(self, board: List[List[str]]) -> None:\n n = 9\n \n \n def isValid(row, col, ch):\n row, col = int(row), int(col)\n \n for i in range(9):\n \n if board[i][col] == ch:\n return False\n if board[row][i] == ch:\n return False\n \n if board[3*(row//3) + i//3][3*(col//3) + i%3] == ch:\n return False\n \n return True\n \n def solve(row, col):\n if row == n:\n return True\n if col == n:\n return solve(row+1, 0)\n \n if board[row][col] == ".":\n for i in range(1, 10):\n if isValid(row, col, str(i)):\n board[row][col] = str(i)\n \n if solve(row, col + 1):\n return True\n else:\n board[row][col] = "."\n return False\n else:\n return solve(row, col + 1)\n \n \n \n solve(0, 0)\n\t\t\n\t\t#do upvote if it helps. | 73 | Write a program to solve a Sudoku puzzle by filling the empty cells.
A sudoku solution must satisfy **all of the following rules**:
1. Each of the digits `1-9` must occur exactly once in each row.
2. Each of the digits `1-9` must occur exactly once in each column.
3. Each of the digits `1-9` must occur exactly once in each of the 9 `3x3` sub-boxes of the grid.
The `'.'` character indicates empty cells.
**Example 1:**
**Input:** board = \[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\],\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\],\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\],\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\],\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\],\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\],\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\],\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\],\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** \[\[ "5 ", "3 ", "4 ", "6 ", "7 ", "8 ", "9 ", "1 ", "2 "\],\[ "6 ", "7 ", "2 ", "1 ", "9 ", "5 ", "3 ", "4 ", "8 "\],\[ "1 ", "9 ", "8 ", "3 ", "4 ", "2 ", "5 ", "6 ", "7 "\],\[ "8 ", "5 ", "9 ", "7 ", "6 ", "1 ", "4 ", "2 ", "3 "\],\[ "4 ", "2 ", "6 ", "8 ", "5 ", "3 ", "7 ", "9 ", "1 "\],\[ "7 ", "1 ", "3 ", "9 ", "2 ", "4 ", "8 ", "5 ", "6 "\],\[ "9 ", "6 ", "1 ", "5 ", "3 ", "7 ", "2 ", "8 ", "4 "\],\[ "2 ", "8 ", "7 ", "4 ", "1 ", "9 ", "6 ", "3 ", "5 "\],\[ "3 ", "4 ", "5 ", "2 ", "8 ", "6 ", "1 ", "7 ", "9 "\]\]
**Explanation:** The input board is shown above and the only valid solution is shown below:
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit or `'.'`.
* It is **guaranteed** that the input board has only one solution. | null |
Readable and intuitive solution in python3. | sudoku-solver | 0 | 1 | # Intuition\nMaintain a row array and a column array to store theh values that are entered in a row. Also, maintain an array for the numbers entered in the current block.\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nStart looping from 1 to 9 for each empty cells and check if the number exists in a row, column or current block, if it doesn\'t, consider that number as a possible solution and go to the next cell. \nChecking the current block number is a bit tricky but can be resolved using simlpe arithmetic (see comment in code below).\nIf in the future, the number considered turns out to be a deadend, remove the considered number from the row, col and current block array and move to the next number.\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(9^2)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(9^2)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def solveSudoku(self, board: List[List[str]]) -> None:\n row = [set() for _ in range(9)]\n col = [set() for _ in range(9)]\n cur = [set() for _ in range(9)]\n for i in range(9):\n for j in range(9):\n if board[i][j] != ".":\n val = int(board[i][j])\n row[i].add(val)\n col[j].add(val)\n# simple arithmetic formula to get the current block id\n cur_id = i//3 * 3 + j//3\n cur[cur_id].add(val)\n \n def bt(i, j):\n nonlocal solved \n if i == 9:\n solved = True\n return\n i1 = i+(j+1)//9\n j1 = (j+1)%9\n if board[i][j] != ".":\n bt(i1, j1)\n else:\n for num in range(1, 10):\n# reusing the same current block formula\n cur_id = i//3 * 3 + j//3\n if num not in row[i] and num not in col[j] and num not in cur[cur_id]:\n row[i].add(num)\n col[j].add(num)\n cur[cur_id].add(num)\n board[i][j] = str(num)\n bt(i1, j1)\n if solved == False:\n row[i].remove(num)\n col[j].remove(num)\n cur[cur_id].remove(num)\n board[i][j] = \'.\'\n solved = False\n bt(0, 0)\n``` | 2 | Write a program to solve a Sudoku puzzle by filling the empty cells.
A sudoku solution must satisfy **all of the following rules**:
1. Each of the digits `1-9` must occur exactly once in each row.
2. Each of the digits `1-9` must occur exactly once in each column.
3. Each of the digits `1-9` must occur exactly once in each of the 9 `3x3` sub-boxes of the grid.
The `'.'` character indicates empty cells.
**Example 1:**
**Input:** board = \[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\],\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\],\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\],\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\],\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\],\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\],\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\],\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\],\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** \[\[ "5 ", "3 ", "4 ", "6 ", "7 ", "8 ", "9 ", "1 ", "2 "\],\[ "6 ", "7 ", "2 ", "1 ", "9 ", "5 ", "3 ", "4 ", "8 "\],\[ "1 ", "9 ", "8 ", "3 ", "4 ", "2 ", "5 ", "6 ", "7 "\],\[ "8 ", "5 ", "9 ", "7 ", "6 ", "1 ", "4 ", "2 ", "3 "\],\[ "4 ", "2 ", "6 ", "8 ", "5 ", "3 ", "7 ", "9 ", "1 "\],\[ "7 ", "1 ", "3 ", "9 ", "2 ", "4 ", "8 ", "5 ", "6 "\],\[ "9 ", "6 ", "1 ", "5 ", "3 ", "7 ", "2 ", "8 ", "4 "\],\[ "2 ", "8 ", "7 ", "4 ", "1 ", "9 ", "6 ", "3 ", "5 "\],\[ "3 ", "4 ", "5 ", "2 ", "8 ", "6 ", "1 ", "7 ", "9 "\]\]
**Explanation:** The input board is shown above and the only valid solution is shown below:
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit or `'.'`.
* It is **guaranteed** that the input board has only one solution. | null |
Superb Logic Backtracking | sudoku-solver | 0 | 1 | # Backtracking\n```\nclass Solution:\n def solveSudoku(self, grid: List[List[str]]) -> None:\n return self.solve(grid)\n def solve(self,grid):\n for i in range(len(grid)):\n for j in range(len(grid[0])):\n if grid[i][j]==".":\n for c in range(1,len(grid)+1):\n c=str(c)\n if self.isvalid(i,j,c,grid):\n grid[i][j]=c\n if self.solve(grid)==True:\n return True\n else:\n grid[i][j]="."\n return False\n return True\n def isvalid(self,row,col,c,grid):\n for i in range(len(grid)):\n if grid[row][i]==c:\n return False\n if grid[i][col]==c:\n return False\n if grid[(3*(row//3)+i//3)][3*(col//3)+i%3]==c:\n return False\n return True\n```\n# please upvote me it would encourage me alot\n | 8 | Write a program to solve a Sudoku puzzle by filling the empty cells.
A sudoku solution must satisfy **all of the following rules**:
1. Each of the digits `1-9` must occur exactly once in each row.
2. Each of the digits `1-9` must occur exactly once in each column.
3. Each of the digits `1-9` must occur exactly once in each of the 9 `3x3` sub-boxes of the grid.
The `'.'` character indicates empty cells.
**Example 1:**
**Input:** board = \[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\],\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\],\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\],\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\],\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\],\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\],\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\],\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\],\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** \[\[ "5 ", "3 ", "4 ", "6 ", "7 ", "8 ", "9 ", "1 ", "2 "\],\[ "6 ", "7 ", "2 ", "1 ", "9 ", "5 ", "3 ", "4 ", "8 "\],\[ "1 ", "9 ", "8 ", "3 ", "4 ", "2 ", "5 ", "6 ", "7 "\],\[ "8 ", "5 ", "9 ", "7 ", "6 ", "1 ", "4 ", "2 ", "3 "\],\[ "4 ", "2 ", "6 ", "8 ", "5 ", "3 ", "7 ", "9 ", "1 "\],\[ "7 ", "1 ", "3 ", "9 ", "2 ", "4 ", "8 ", "5 ", "6 "\],\[ "9 ", "6 ", "1 ", "5 ", "3 ", "7 ", "2 ", "8 ", "4 "\],\[ "2 ", "8 ", "7 ", "4 ", "1 ", "9 ", "6 ", "3 ", "5 "\],\[ "3 ", "4 ", "5 ", "2 ", "8 ", "6 ", "1 ", "7 ", "9 "\]\]
**Explanation:** The input board is shown above and the only valid solution is shown below:
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit or `'.'`.
* It is **guaranteed** that the input board has only one solution. | null |
Python || Striver's Solution || Backtracking || Easy | sudoku-solver | 0 | 1 | ```\nclass Solution:\n def solveSudoku(self, board: List[List[str]]) -> None:\n def safe(row,col,k,board): #function to check whether it is safe to insert a no. in board or not\n for i in range(9):\n if board[row][i]==k: # for checking element in same row\n return False\n if board[i][col]==k: # for checking element in same column\n return False\n if board[3*(row//3)+i//3][3*(col//3)+i%3]==k: # for checking element in same box\n return False\n return True\n def solve(board):\n for i in range(9):\n for j in range(9):\n if board[i][j]==\'.\':\n for k in range(1,10):\n k=str(k)\n if safe(i,j,k,board):\n board[i][j]=k\n if solve(board):\n return True\n board[i][j]=\'.\'\n return False # if it is not possible to insert any particular value at that cell\n return True # if all the values are filled then it will return True\n solve(board)\n```\n**An upvote will be encouraging** | 4 | Write a program to solve a Sudoku puzzle by filling the empty cells.
A sudoku solution must satisfy **all of the following rules**:
1. Each of the digits `1-9` must occur exactly once in each row.
2. Each of the digits `1-9` must occur exactly once in each column.
3. Each of the digits `1-9` must occur exactly once in each of the 9 `3x3` sub-boxes of the grid.
The `'.'` character indicates empty cells.
**Example 1:**
**Input:** board = \[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\],\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\],\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\],\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\],\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\],\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\],\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\],\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\],\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** \[\[ "5 ", "3 ", "4 ", "6 ", "7 ", "8 ", "9 ", "1 ", "2 "\],\[ "6 ", "7 ", "2 ", "1 ", "9 ", "5 ", "3 ", "4 ", "8 "\],\[ "1 ", "9 ", "8 ", "3 ", "4 ", "2 ", "5 ", "6 ", "7 "\],\[ "8 ", "5 ", "9 ", "7 ", "6 ", "1 ", "4 ", "2 ", "3 "\],\[ "4 ", "2 ", "6 ", "8 ", "5 ", "3 ", "7 ", "9 ", "1 "\],\[ "7 ", "1 ", "3 ", "9 ", "2 ", "4 ", "8 ", "5 ", "6 "\],\[ "9 ", "6 ", "1 ", "5 ", "3 ", "7 ", "2 ", "8 ", "4 "\],\[ "2 ", "8 ", "7 ", "4 ", "1 ", "9 ", "6 ", "3 ", "5 "\],\[ "3 ", "4 ", "5 ", "2 ", "8 ", "6 ", "1 ", "7 ", "9 "\]\]
**Explanation:** The input board is shown above and the only valid solution is shown below:
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit or `'.'`.
* It is **guaranteed** that the input board has only one solution. | null |
Easy solution using recursion, backtracking and math | sudoku-solver | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n bool isValid(vector<vector<char>> &board, int row, int col, char c) {\n for (int i = 0; i < 9; i++) {\n if (board[i][col] == c)\n return false;\n if (board[row][i] == c)\n return false;\n if (board[3 * (row / 3) + i / 3][3 * (col / 3) + i % 3] == c)\n return false;\n }\n return true;\n }\n\n bool solve(vector<vector<char>> &board) {\n for (int i = 0; i < board.size(); i++) {\n for (int j = 0; j < board[0].size(); j++) {\n if (board[i][j] == \'.\') {\n for (char c = \'1\'; c <= \'9\'; c++) {\n if (isValid(board, i, j, c)) {\n board[i][j] = c;\n if (solve(board))\n return true;\n else\n board[i][j] = \'.\';\n }\n }\n return false;\n }\n }\n }\n return true;\n }\n \n void solveSudoku(vector<vector<char>> &board) {\n solve(board);\n }\n};\n\n```\n```JAVA []\nclass Solution {\n\n public boolean isValid(char[][] board, int row, int col, char c) {\n for (int i = 0; i < 9; i++) {\n if (board[i][col] == c) return false;\n if (board[row][i] == c) return false;\n if (board[3 * (row / 3) + i / 3][3 * (col / 3) + i % 3] == c) return false;\n }\n return true;\n }\n\n public boolean solve(char[][] board) {\n for (int i = 0; i < 9; i++) {\n for (int j = 0; j < 9; j++) {\n if (board[i][j] == \'.\') {\n for (char c = \'1\'; c <= \'9\'; c++) {\n if (isValid(board, i, j, c)) {\n board[i][j] = c;\n if (solve(board)) return true;\n else board[i][j] = \'.\';\n }\n }\n return false;\n }\n }\n }\n return true;\n }\n\n public void solveSudoku(char[][] board) {\n solve(board);\n }\n}\n\n```\n```Python []\nclass Solution:\n def solveSudoku(self, board: List[List[str]]) -> None:\n def isValid(board, row, col, c):\n for i in range(9):\n if board[i][col] == c:\n return False\n if board[row][i] == c:\n return False\n row_index = 3 * (row // 3) + i // 3\n col_index = 3 * (col // 3) + i % 3\n if board[row_index][col_index] == c:\n return False\n return True\n\n def solve(board):\n for row in range(9):\n for col in range(9):\n if board[row][col] == ".":\n for c in range(1, 10):\n if isValid(board, row, col, chr(c + ord("0"))):\n board[row][col] = chr(c + ord("0"))\n if solve(board):\n return True\n else:\n board[row][col] = "."\n return False\n return True\n\n solve(board)\n\n\n\n```\n | 6 | Write a program to solve a Sudoku puzzle by filling the empty cells.
A sudoku solution must satisfy **all of the following rules**:
1. Each of the digits `1-9` must occur exactly once in each row.
2. Each of the digits `1-9` must occur exactly once in each column.
3. Each of the digits `1-9` must occur exactly once in each of the 9 `3x3` sub-boxes of the grid.
The `'.'` character indicates empty cells.
**Example 1:**
**Input:** board = \[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\],\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\],\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\],\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\],\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\],\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\],\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\],\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\],\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** \[\[ "5 ", "3 ", "4 ", "6 ", "7 ", "8 ", "9 ", "1 ", "2 "\],\[ "6 ", "7 ", "2 ", "1 ", "9 ", "5 ", "3 ", "4 ", "8 "\],\[ "1 ", "9 ", "8 ", "3 ", "4 ", "2 ", "5 ", "6 ", "7 "\],\[ "8 ", "5 ", "9 ", "7 ", "6 ", "1 ", "4 ", "2 ", "3 "\],\[ "4 ", "2 ", "6 ", "8 ", "5 ", "3 ", "7 ", "9 ", "1 "\],\[ "7 ", "1 ", "3 ", "9 ", "2 ", "4 ", "8 ", "5 ", "6 "\],\[ "9 ", "6 ", "1 ", "5 ", "3 ", "7 ", "2 ", "8 ", "4 "\],\[ "2 ", "8 ", "7 ", "4 ", "1 ", "9 ", "6 ", "3 ", "5 "\],\[ "3 ", "4 ", "5 ", "2 ", "8 ", "6 ", "1 ", "7 ", "9 "\]\]
**Explanation:** The input board is shown above and the only valid solution is shown below:
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit or `'.'`.
* It is **guaranteed** that the input board has only one solution. | null |
Python Iterative backtracking solution.. Easy to understand | sudoku-solver | 0 | 1 | \n# Code\n```\nclass Solution:\n \n def solveSudoku(self, board: List[List[str]]) -> None:\n row = { i:[0]*10 for i in range(0,9) }\n col = { i:[0]*10 for i in range(0,9) }\n box = { i:[0]*10 for i in range(0,9) }\n n,m = len(board),len(board[0])\n for i in range(0,n):\n for j in range(0,m):\n box_index = 3*(i//3) + j//3 \n c = ord(board[i][j][0]) - ord(\'0\')\n if( c < 0 or c > 9 ):\n board[i][j] = 0\n else: \n board[i][j] = c ;\n row[i][c] = 1\n col[j][c] = 1\n box[box_index][c] = 1\n\n \n def rec(i,j):\n if( board[i][j] > 0 and board[i][j] <= 9 ):\n if( j < 8 ):\n if( rec(i,j+1)):\n return True\n elif( i < 8 ):\n if( rec(i+1,0) ):\n return True \n elif( i == 8 and j == 8 ):\n return True ;\n else:\n box_index = 3*(i//3) + j//3 \n for k in range(1,10):\n if( row[i][k] == col[j][k] == box[box_index][k] == 0 ):\n row[i][k] = col[j][k] = box[box_index][k] = 1\n board[i][j] = k \n if( j < 8 ):\n if(rec(i,j+1)):\n return True;\n elif( i < 8 ):\n if( rec(i+1,0) ):\n return True ;\n else:\n return True;\n board[i][j] = 0 \n row[i][k] = col[j][k] = box[box_index][k] = 0\n \n return False;\n \n rec(0,0)\n\n \n for i in range(0,9):\n for j in range(0,9):\n board[i][j] = chr(board[i][j]+ord(\'0\'))\n\n\n return board;\n\n \n\n``` | 3 | Write a program to solve a Sudoku puzzle by filling the empty cells.
A sudoku solution must satisfy **all of the following rules**:
1. Each of the digits `1-9` must occur exactly once in each row.
2. Each of the digits `1-9` must occur exactly once in each column.
3. Each of the digits `1-9` must occur exactly once in each of the 9 `3x3` sub-boxes of the grid.
The `'.'` character indicates empty cells.
**Example 1:**
**Input:** board = \[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\],\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\],\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\],\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\],\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\],\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\],\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\],\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\],\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** \[\[ "5 ", "3 ", "4 ", "6 ", "7 ", "8 ", "9 ", "1 ", "2 "\],\[ "6 ", "7 ", "2 ", "1 ", "9 ", "5 ", "3 ", "4 ", "8 "\],\[ "1 ", "9 ", "8 ", "3 ", "4 ", "2 ", "5 ", "6 ", "7 "\],\[ "8 ", "5 ", "9 ", "7 ", "6 ", "1 ", "4 ", "2 ", "3 "\],\[ "4 ", "2 ", "6 ", "8 ", "5 ", "3 ", "7 ", "9 ", "1 "\],\[ "7 ", "1 ", "3 ", "9 ", "2 ", "4 ", "8 ", "5 ", "6 "\],\[ "9 ", "6 ", "1 ", "5 ", "3 ", "7 ", "2 ", "8 ", "4 "\],\[ "2 ", "8 ", "7 ", "4 ", "1 ", "9 ", "6 ", "3 ", "5 "\],\[ "3 ", "4 ", "5 ", "2 ", "8 ", "6 ", "1 ", "7 ", "9 "\]\]
**Explanation:** The input board is shown above and the only valid solution is shown below:
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit or `'.'`.
* It is **guaranteed** that the input board has only one solution. | null |
[Python3] - Straightforward and Readable Solution | sudoku-solver | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe easiest Method is just trial and error. We do this by finding and empty cell and checking which numbers are available for this cell. Then we input these numbers one by one and traverse deeper continuing this approach recursively until we meet one of the following two cases:\n\n1) The grid does not contain empty cells anymore. This means we found a valid solution.\n2) We have an empty cell, but there are no available numbers any more.\n\nIn case of 2) we need to backtrack through the recursion and undo the changes we made to the grid.\n\nWe could make this a little fast by starting from position whith the least available numbers.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nIn my approach I tried to keep it readable by splitting the work into small and independent functions.\n\nThey are named in a readable manner, so I think one can see the purpose of each of the functions.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nTime complexity is O(N*M) but I\'m not entirely sure about this one, so I stand corrected.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space is taken by the recursion stack which is O(N*M) in the worst case.\n\n# Code\n```Python\nclass Solution:\n\n # make a set of possible characters\n possible = set(str(nr) for nr in range(1,10))\n\n def solveSudoku(self, board: List[List[str]]) -> None:\n """\n Do not return anything, modify board in-place instead.\n """\n \n # save the board\n self.board = board\n self.dfs()\n \n def dfs(self):\n\n # find the next empty cell\n rx, cx, complete = self.findEmpty()\n\n # check whether there are empty cells\n if complete:\n return True\n \n # get the available numbers\n available = self.getPossible(rx, cx)\n\n # check whether we have available numbers\n if not available:\n return False\n \n # go through available numbers and try them\n for number in available:\n\n # set the number\n self.board[rx][cx] = number\n\n # check whether it works\n if self.dfs():\n return True\n \n # reset the number\n self.board[rx][cx] = "."\n \n return False\n\n def findEmpty(self):\n # find the next free position and if all are filled we can return True\n for rx in range(len(self.board)):\n for cx in range(len(self.board[0])):\n if self.board[rx][cx] == \'.\':\n return rx, cx, False\n return -1, -1, True\n\n def getPossible(self, rx, cx):\n return self.possible - self.getBox(rx, cx) - self.getRow(rx, cx) - self.getCol(rx, cx)\n\n def getRow(self, rx, cx):\n return set(self.board[rx])\n\n def getCol(self, rx, cx):\n return set([row[cx] for row in self.board])\n\n def getBox(self, rx, cx):\n result = set()\n for rx in range(rx // 3 * 3, rx // 3 * 3 + 3):\n result.update(self.board[rx][cx // 3 * 3:cx // 3 * 3 + 3])\n return result\n \n``` | 6 | Write a program to solve a Sudoku puzzle by filling the empty cells.
A sudoku solution must satisfy **all of the following rules**:
1. Each of the digits `1-9` must occur exactly once in each row.
2. Each of the digits `1-9` must occur exactly once in each column.
3. Each of the digits `1-9` must occur exactly once in each of the 9 `3x3` sub-boxes of the grid.
The `'.'` character indicates empty cells.
**Example 1:**
**Input:** board = \[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\],\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\],\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\],\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\],\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\],\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\],\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\],\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\],\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** \[\[ "5 ", "3 ", "4 ", "6 ", "7 ", "8 ", "9 ", "1 ", "2 "\],\[ "6 ", "7 ", "2 ", "1 ", "9 ", "5 ", "3 ", "4 ", "8 "\],\[ "1 ", "9 ", "8 ", "3 ", "4 ", "2 ", "5 ", "6 ", "7 "\],\[ "8 ", "5 ", "9 ", "7 ", "6 ", "1 ", "4 ", "2 ", "3 "\],\[ "4 ", "2 ", "6 ", "8 ", "5 ", "3 ", "7 ", "9 ", "1 "\],\[ "7 ", "1 ", "3 ", "9 ", "2 ", "4 ", "8 ", "5 ", "6 "\],\[ "9 ", "6 ", "1 ", "5 ", "3 ", "7 ", "2 ", "8 ", "4 "\],\[ "2 ", "8 ", "7 ", "4 ", "1 ", "9 ", "6 ", "3 ", "5 "\],\[ "3 ", "4 ", "5 ", "2 ", "8 ", "6 ", "1 ", "7 ", "9 "\]\]
**Explanation:** The input board is shown above and the only valid solution is shown below:
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit or `'.'`.
* It is **guaranteed** that the input board has only one solution. | null |
🐍 97% faster || Clean & Concise || Well-Explained 📌📌 | sudoku-solver | 0 | 1 | ## IDEA:\n*The simple idea here is to fill a position and take the updated board as the new board and check if this new board has a possible solution.\nIf there is no valid solution for our new board we revert back to our previous state of the board and try by filling the next possible solution.*\n\nChecking if a value is valid in the respective empty slot:\nBefore intialising a value to our empty spot we need to check if that value is valid at that spot. For that we should check three cases:\n1) If value is already present in the row\n2) If value is already presnt in the column\n3) If value is already presnt in the smaller(3*3)box .(This is a bit tricky)\n\n****\nFeel free to ask and get your answer within 24hrs if you have any doubts. \uD83E\uDD16\n**Upvote** if you got any help !! \uD83E\uDD1E\n****\n\n\'\'\'\n\n\tfrom collections import defaultdict,deque\n\tclass Solution:\n\t\tdef solveSudoku(self, board: List[List[str]]) -> None:\n \n rows,cols,block,seen = defaultdict(set),defaultdict(set),defaultdict(set),deque([])\n for i in range(9):\n for j in range(9):\n if board[i][j]!=".":\n rows[i].add(board[i][j])\n cols[j].add(board[i][j])\n block[(i//3,j//3)].add(board[i][j])\n else:\n seen.append((i,j))\n \n def dfs():\n if not seen:\n return True\n \n r,c = seen[0]\n t = (r//3,c//3)\n for n in {\'1\',\'2\',\'3\',\'4\',\'5\',\'6\',\'7\',\'8\',\'9\'}:\n if n not in rows[r] and n not in cols[c] and n not in block[t]:\n board[r][c]=n\n rows[r].add(n)\n cols[c].add(n)\n block[t].add(n)\n seen.popleft()\n if dfs():\n return True\n else:\n board[r][c]="."\n rows[r].discard(n)\n cols[c].discard(n)\n block[t].discard(n)\n seen.appendleft((r,c))\n return False\n \n dfs()\n\n### Thanks \uD83E\uDD17 | 33 | Write a program to solve a Sudoku puzzle by filling the empty cells.
A sudoku solution must satisfy **all of the following rules**:
1. Each of the digits `1-9` must occur exactly once in each row.
2. Each of the digits `1-9` must occur exactly once in each column.
3. Each of the digits `1-9` must occur exactly once in each of the 9 `3x3` sub-boxes of the grid.
The `'.'` character indicates empty cells.
**Example 1:**
**Input:** board = \[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\],\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\],\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\],\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\],\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\],\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\],\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\],\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\],\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** \[\[ "5 ", "3 ", "4 ", "6 ", "7 ", "8 ", "9 ", "1 ", "2 "\],\[ "6 ", "7 ", "2 ", "1 ", "9 ", "5 ", "3 ", "4 ", "8 "\],\[ "1 ", "9 ", "8 ", "3 ", "4 ", "2 ", "5 ", "6 ", "7 "\],\[ "8 ", "5 ", "9 ", "7 ", "6 ", "1 ", "4 ", "2 ", "3 "\],\[ "4 ", "2 ", "6 ", "8 ", "5 ", "3 ", "7 ", "9 ", "1 "\],\[ "7 ", "1 ", "3 ", "9 ", "2 ", "4 ", "8 ", "5 ", "6 "\],\[ "9 ", "6 ", "1 ", "5 ", "3 ", "7 ", "2 ", "8 ", "4 "\],\[ "2 ", "8 ", "7 ", "4 ", "1 ", "9 ", "6 ", "3 ", "5 "\],\[ "3 ", "4 ", "5 ", "2 ", "8 ", "6 ", "1 ", "7 ", "9 "\]\]
**Explanation:** The input board is shown above and the only valid solution is shown below:
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit or `'.'`.
* It is **guaranteed** that the input board has only one solution. | null |
[Fastest Solution Explained] O(n)time complexity O(n)space complexity | sudoku-solver | 1 | 1 | (Note: This is part of a series of Leetcode solution explanations. If you like this solution or find it useful, ***please upvote*** this post.)\n***Take care brother, peace, love!***\n\n```\n```\n\nThe best result for the code below is ***0ms / 38.2MB*** (beats 92.04% / 24.00%).\n* *** Java ***\n\n```\n\nclass Solution {\n private static final char EMPTY_ENTRY = \'.\';\n\n/*\n Driver function to kick off the recursion\n*/\npublic static boolean solveSudoku(char[][] board){\n return solveSudokuCell(0, 0, board);\n}\n\n/*\n This function chooses a placement for the cell at (row, col)\n and continues solving based on the rules we define.\n \n Our strategy:\n We will start at row 0.\n We will solve every column in that row.\n When we reach the last column we move to the next row.\n If this is past the last row (row == board.length) we are done.\n The whole board has been solved.\n*/\nprivate static boolean solveSudokuCell(int row, int col, char[][] board) {\n\n /*\n Have we finished placements in all columns for\n the row we are working on?\n */\n if (col == board[row].length){\n\n /*\n Yes. Reset to col 0 and advance the row by 1.\n We will work on the next row.\n */\n col = 0;\n row++;\n\n /*\n Have we completed placements in all rows? If so then we are done.\n If not, drop through to the logic below and keep solving things.\n */\n if (row == board.length){\n return true; // Entire board has been filled without conflict.\n }\n\n }\n\n // Skip non-empty entries. They already have a value in them.\n if (board[row][col] != EMPTY_ENTRY) {\n return solveSudokuCell(row, col + 1, board);\n }\n\n /*\n Try all values 1 through 9 in the cell at (row, col).\n Recurse on the placement if it doesn\'t break the constraints of Sudoku.\n */\n for (int value = 1; value <= board.length; value++) {\n\n char charToPlace = (char) (value + \'0\'); // convert int value to char\n\n /*\n Apply constraints. We will only add the value to the cell if\n adding it won\'t cause us to break sudoku rules.\n */\n if (canPlaceValue(board, row, col, charToPlace)) {\n board[row][col] = charToPlace;\n if (solveSudokuCell(row, col + 1, board)) { // recurse with our VALID placement\n return true;\n }\n }\n\n }\n\n /*\n Undo assignment to this cell. No values worked in it meaning that\n previous states put us in a position we cannot solve from. Hence,\n we backtrack by returning "false" to our caller.\n */\n board[row][col] = EMPTY_ENTRY;\n return false; // No valid placement was found, this path is faulty, return false\n}\n\n/*\n Will the placement at (row, col) break the Sudoku properties?\n*/\nprivate static boolean canPlaceValue(char[][] board, int row, int col, char charToPlace) {\n\n // Check column constraint. For each row, we do a check on column "col".\n for (char[] element : board) {\n if (charToPlace == element[col]){\n return false;\n }\n }\n\n // Check row constraint. For each column in row "row", we do a check.\n for (int i = 0; i < board.length; i++) {\n if (charToPlace == board[row][i]) {\n return false;\n }\n }\n\n /*\n Check region constraints.\n \n In a 9 x 9 board, we will have 9 sub-boxes (3 rows of 3 sub-boxes).\n \n The "I" tells us that we are in the Ith sub-box row. (there are 3 sub-box rows)\n The "J" tells us that we are in the Jth sub-box column. (there are 3 sub-box columns)\n \n I tried to think of better variable names for like 20 minutes but couldn\'t so just left\n I and J.\n \n Integer properties will truncate the decimal place so we just know the sub-box number we are in.\n Each coordinate we touch will be found by an offset from topLeftOfSubBoxRow and topLeftOfSubBoxCol.\n */\n int regionSize = (int) Math.sqrt(board.length); // gives us the size of a sub-box\n\n int I = row / regionSize;\n int J = col / regionSize;\n\n /*\n This multiplication takes us to the EXACT top left of the sub-box. We keep the (row, col)\n of these values because it is important. It lets us traverse the sub-box with our double for loop.\n */\n int topLeftOfSubBoxRow = regionSize * I; // the row of the top left of the block\n int topLeftOfSubBoxCol = regionSize * J; // the column of the tol left of the block\n\n for (int i = 0; i < regionSize; i++) {\n for (int j = 0; j < regionSize; j++) {\n\n /*\n i and j just define our offsets from topLeftOfBlockRow\n and topLeftOfBlockCol respectively\n */\n if (charToPlace == board[topLeftOfSubBoxRow + i][topLeftOfSubBoxCol + j]) {\n return false;\n }\n\n }\n }\n\n return true; // placement is valid\n}\n}\n\n```\n\n```\n```\n\n```\n```\n\n***"Open your eyes. Expect us." - \uD835\uDCD0\uD835\uDCF7\uD835\uDCF8\uD835\uDCF7\uD835\uDD02\uD835\uDCF6\uD835\uDCF8\uD835\uDCFE\uD835\uDCFC***\n | 8 | Write a program to solve a Sudoku puzzle by filling the empty cells.
A sudoku solution must satisfy **all of the following rules**:
1. Each of the digits `1-9` must occur exactly once in each row.
2. Each of the digits `1-9` must occur exactly once in each column.
3. Each of the digits `1-9` must occur exactly once in each of the 9 `3x3` sub-boxes of the grid.
The `'.'` character indicates empty cells.
**Example 1:**
**Input:** board = \[\[ "5 ", "3 ", ". ", ". ", "7 ", ". ", ". ", ". ", ". "\],\[ "6 ", ". ", ". ", "1 ", "9 ", "5 ", ". ", ". ", ". "\],\[ ". ", "9 ", "8 ", ". ", ". ", ". ", ". ", "6 ", ". "\],\[ "8 ", ". ", ". ", ". ", "6 ", ". ", ". ", ". ", "3 "\],\[ "4 ", ". ", ". ", "8 ", ". ", "3 ", ". ", ". ", "1 "\],\[ "7 ", ". ", ". ", ". ", "2 ", ". ", ". ", ". ", "6 "\],\[ ". ", "6 ", ". ", ". ", ". ", ". ", "2 ", "8 ", ". "\],\[ ". ", ". ", ". ", "4 ", "1 ", "9 ", ". ", ". ", "5 "\],\[ ". ", ". ", ". ", ". ", "8 ", ". ", ". ", "7 ", "9 "\]\]
**Output:** \[\[ "5 ", "3 ", "4 ", "6 ", "7 ", "8 ", "9 ", "1 ", "2 "\],\[ "6 ", "7 ", "2 ", "1 ", "9 ", "5 ", "3 ", "4 ", "8 "\],\[ "1 ", "9 ", "8 ", "3 ", "4 ", "2 ", "5 ", "6 ", "7 "\],\[ "8 ", "5 ", "9 ", "7 ", "6 ", "1 ", "4 ", "2 ", "3 "\],\[ "4 ", "2 ", "6 ", "8 ", "5 ", "3 ", "7 ", "9 ", "1 "\],\[ "7 ", "1 ", "3 ", "9 ", "2 ", "4 ", "8 ", "5 ", "6 "\],\[ "9 ", "6 ", "1 ", "5 ", "3 ", "7 ", "2 ", "8 ", "4 "\],\[ "2 ", "8 ", "7 ", "4 ", "1 ", "9 ", "6 ", "3 ", "5 "\],\[ "3 ", "4 ", "5 ", "2 ", "8 ", "6 ", "1 ", "7 ", "9 "\]\]
**Explanation:** The input board is shown above and the only valid solution is shown below:
**Constraints:**
* `board.length == 9`
* `board[i].length == 9`
* `board[i][j]` is a digit or `'.'`.
* It is **guaranteed** that the input board has only one solution. | null |
Easy python solution: recursive approach | count-and-say | 0 | 1 | ```\nclass Solution:\n def countAndSay(self, n: int) -> str:\n if n==1:\n return "1"\n x=self.countAndSay(n-1)\n s=""\n y=x[0]\n ct=1\n for i in range(1,len(x)):\n if x[i]==y:\n ct+=1\n else:\n s+=str(ct)\n s+=str(y)\n y=x[i]\n ct=1\n s+=str(ct)\n s+=str(y)\n return s\n``` | 24 | The **count-and-say** sequence is a sequence of digit strings defined by the recursive formula:
* `countAndSay(1) = "1 "`
* `countAndSay(n)` is the way you would "say " the digit string from `countAndSay(n-1)`, which is then converted into a different digit string.
To determine how you "say " a digit string, split it into the **minimal** number of substrings such that each substring contains exactly **one** unique digit. Then for each substring, say the number of digits, then say the digit. Finally, concatenate every said digit.
For example, the saying and conversion for digit string `"3322251 "`:
Given a positive integer `n`, return _the_ `nth` _term of the **count-and-say** sequence_.
**Example 1:**
**Input:** n = 1
**Output:** "1 "
**Explanation:** This is the base case.
**Example 2:**
**Input:** n = 4
**Output:** "1211 "
**Explanation:**
countAndSay(1) = "1 "
countAndSay(2) = say "1 " = one 1 = "11 "
countAndSay(3) = say "11 " = two 1's = "21 "
countAndSay(4) = say "21 " = one 2 + one 1 = "12 " + "11 " = "1211 "
**Constraints:**
* `1 <= n <= 30` | The following are the terms from n=1 to n=10 of the count-and-say sequence:
1. 1
2. 11
3. 21
4. 1211
5. 111221
6. 312211
7. 13112221
8. 1113213211
9. 31131211131221
10. 13211311123113112211 To generate the nth term, just count and say the n-1th term. |
Easy python solution: recursive approach | count-and-say | 0 | 1 | ```\nclass Solution:\n def countAndSay(self, n: int) -> str:\n if n==1:\n return "1"\n x=self.countAndSay(n-1)\n s=""\n y=x[0]\n ct=1\n for i in range(1,len(x)):\n if x[i]==y:\n ct+=1\n else:\n s+=str(ct)\n s+=str(y)\n y=x[i]\n ct=1\n s+=str(ct)\n s+=str(y)\n return s\n``` | 24 | The **count-and-say** sequence is a sequence of digit strings defined by the recursive formula:
* `countAndSay(1) = "1 "`
* `countAndSay(n)` is the way you would "say " the digit string from `countAndSay(n-1)`, which is then converted into a different digit string.
To determine how you "say " a digit string, split it into the **minimal** number of substrings such that each substring contains exactly **one** unique digit. Then for each substring, say the number of digits, then say the digit. Finally, concatenate every said digit.
For example, the saying and conversion for digit string `"3322251 "`:
Given a positive integer `n`, return _the_ `nth` _term of the **count-and-say** sequence_.
**Example 1:**
**Input:** n = 1
**Output:** "1 "
**Explanation:** This is the base case.
**Example 2:**
**Input:** n = 4
**Output:** "1211 "
**Explanation:**
countAndSay(1) = "1 "
countAndSay(2) = say "1 " = one 1 = "11 "
countAndSay(3) = say "11 " = two 1's = "21 "
countAndSay(4) = say "21 " = one 2 + one 1 = "12 " + "11 " = "1211 "
**Constraints:**
* `1 <= n <= 30` | The following are the terms from n=1 to n=10 of the count-and-say sequence:
1. 1
2. 11
3. 21
4. 1211
5. 111221
6. 312211
7. 13112221
8. 1113213211
9. 31131211131221
10. 13211311123113112211 To generate the nth term, just count and say the n-1th term. |
My Python Solution | count-and-say | 0 | 1 | # Code\n```\nclass Solution:\n def countAndSay(self, n: int) -> str:\n if n==1:return "1"\n s="1"\n while n>1:\n tmp=[]\n st=[]\n cnt=0\n for i in s:\n if st and st[-1]==i:\n cnt+=1\n else:\n if cnt>0:\n tmp.append([st[0],cnt])\n cnt=1\n st=[]\n st.append(i)\n if st and cnt>0:\n tmp.append([st[0],cnt])\n lst=[]\n nstr=""\n for i,j in tmp:\n nstr+=str(i)+str(j)\n s=nstr\n n-=1\n return s[::-1]\n\n\n \n``` | 1 | The **count-and-say** sequence is a sequence of digit strings defined by the recursive formula:
* `countAndSay(1) = "1 "`
* `countAndSay(n)` is the way you would "say " the digit string from `countAndSay(n-1)`, which is then converted into a different digit string.
To determine how you "say " a digit string, split it into the **minimal** number of substrings such that each substring contains exactly **one** unique digit. Then for each substring, say the number of digits, then say the digit. Finally, concatenate every said digit.
For example, the saying and conversion for digit string `"3322251 "`:
Given a positive integer `n`, return _the_ `nth` _term of the **count-and-say** sequence_.
**Example 1:**
**Input:** n = 1
**Output:** "1 "
**Explanation:** This is the base case.
**Example 2:**
**Input:** n = 4
**Output:** "1211 "
**Explanation:**
countAndSay(1) = "1 "
countAndSay(2) = say "1 " = one 1 = "11 "
countAndSay(3) = say "11 " = two 1's = "21 "
countAndSay(4) = say "21 " = one 2 + one 1 = "12 " + "11 " = "1211 "
**Constraints:**
* `1 <= n <= 30` | The following are the terms from n=1 to n=10 of the count-and-say sequence:
1. 1
2. 11
3. 21
4. 1211
5. 111221
6. 312211
7. 13112221
8. 1113213211
9. 31131211131221
10. 13211311123113112211 To generate the nth term, just count and say the n-1th term. |
My Python Solution | count-and-say | 0 | 1 | # Code\n```\nclass Solution:\n def countAndSay(self, n: int) -> str:\n if n==1:return "1"\n s="1"\n while n>1:\n tmp=[]\n st=[]\n cnt=0\n for i in s:\n if st and st[-1]==i:\n cnt+=1\n else:\n if cnt>0:\n tmp.append([st[0],cnt])\n cnt=1\n st=[]\n st.append(i)\n if st and cnt>0:\n tmp.append([st[0],cnt])\n lst=[]\n nstr=""\n for i,j in tmp:\n nstr+=str(i)+str(j)\n s=nstr\n n-=1\n return s[::-1]\n\n\n \n``` | 1 | The **count-and-say** sequence is a sequence of digit strings defined by the recursive formula:
* `countAndSay(1) = "1 "`
* `countAndSay(n)` is the way you would "say " the digit string from `countAndSay(n-1)`, which is then converted into a different digit string.
To determine how you "say " a digit string, split it into the **minimal** number of substrings such that each substring contains exactly **one** unique digit. Then for each substring, say the number of digits, then say the digit. Finally, concatenate every said digit.
For example, the saying and conversion for digit string `"3322251 "`:
Given a positive integer `n`, return _the_ `nth` _term of the **count-and-say** sequence_.
**Example 1:**
**Input:** n = 1
**Output:** "1 "
**Explanation:** This is the base case.
**Example 2:**
**Input:** n = 4
**Output:** "1211 "
**Explanation:**
countAndSay(1) = "1 "
countAndSay(2) = say "1 " = one 1 = "11 "
countAndSay(3) = say "11 " = two 1's = "21 "
countAndSay(4) = say "21 " = one 2 + one 1 = "12 " + "11 " = "1211 "
**Constraints:**
* `1 <= n <= 30` | The following are the terms from n=1 to n=10 of the count-and-say sequence:
1. 1
2. 11
3. 21
4. 1211
5. 111221
6. 312211
7. 13112221
8. 1113213211
9. 31131211131221
10. 13211311123113112211 To generate the nth term, just count and say the n-1th term. |
Finding Combination Sum - Backtracking Approach | (Runtime - beats 80.99%) | 2 Way Decision Tree | combination-sum | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe code aims to find all unique combinations of numbers from the candidates list that sum up to the given target. It uses depth-first search (DFS) to explore different combinations, and it backtracks whenever a combination doesn\'t lead to the target sum.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe approach is to use a recursive DFS algorithm. The function dfs is called with three parameters: `i` (the current candidate index), `curr` (the current combination being formed), and `total` (the current sum of the combination elements). It explores two main cases:\n\n- If the `total` is equal to the `target`, the current combination is a valid solution, so it\'s added to the result.\n- If the `total` exceeds the `target` or the index i is out of bounds, the exploration should stop as this path won\'t lead to a valid solution.\n- For each candidate value, the code either includes it in the current combination (`curr`) or skips to the next candidate (`i+1`). It explores all possibilities recursively, and after each exploration, the code backtracks by removing the last added candidate from the current combination using `curr.pop()`.\n\n# Complexity\n- Time complexity: O(2^n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def combinationSum(self, candidates: List[int], target: int) -> List[List[int]]:\n res = []\n def dfs(i, curr, total):\n if total == target:\n res.append(curr.copy())\n return\n \n if i >= len(candidates) or total > target:\n return\n\n curr.append(candidates[i])\n dfs(i, curr, total + candidates[i])\n curr.pop()\n dfs(i+1, curr, total)\n\n dfs(0, [], 0)\n return res\n``` | 1 | Given an array of **distinct** integers `candidates` and a target integer `target`, return _a list of all **unique combinations** of_ `candidates` _where the chosen numbers sum to_ `target`_._ You may return the combinations in **any order**.
The **same** number may be chosen from `candidates` an **unlimited number of times**. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
The test cases are generated such that the number of unique combinations that sum up to `target` is less than `150` combinations for the given input.
**Example 1:**
**Input:** candidates = \[2,3,6,7\], target = 7
**Output:** \[\[2,2,3\],\[7\]\]
**Explanation:**
2 and 3 are candidates, and 2 + 2 + 3 = 7. Note that 2 can be used multiple times.
7 is a candidate, and 7 = 7.
These are the only two combinations.
**Example 2:**
**Input:** candidates = \[2,3,5\], target = 8
**Output:** \[\[2,2,2,2\],\[2,3,3\],\[3,5\]\]
**Example 3:**
**Input:** candidates = \[2\], target = 1
**Output:** \[\]
**Constraints:**
* `1 <= candidates.length <= 30`
* `2 <= candidates[i] <= 40`
* All elements of `candidates` are **distinct**.
* `1 <= target <= 40` | null |
Beat 95% 48 ms with this solution||Backtracking and Recursive. Don't like and upvote ! | combination-sum | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nTo solve this problem, we need to list all possible combination\n==> Use backtracking and recursive\nWe can decrease runtime further by remove some unused branch e.g sum>target\nFor the distinct personally i always run from min to max\n\nExample\n````\ncandidates=[2,3,6,7]\ntarget=7\ncomb and sum\n[[], 0]\n[[2], 2]\n[[2, 2], 4]\n[[2, 2, 2], 6]\n[[2, 2, 2, 2], 8]\nreturn because sum >target\n[[2, 2, 3], 7]\n[[2, 2, 3, 3], 10]\nreturn because sum >target\n[[2, 2, 6], 10]\nreturn because sum >target\n[[2, 3], 5]\n[[2, 3, 3], 8]\nreturn because sum >target\n[[2, 6], 8]\nreturn because sum >target\n[[3], 3]\n[[3, 3], 6]\n[[3, 3, 3], 9]\nreturn because sum >target\n[[3, 6], 9]\nreturn because sum >target\n[[6], 6]\n[[6, 6], 12]\nreturn because sum >target\n[[7], 7]\n[[7, 7], 14]\nreturn because sum >target\n````\n\n# Complexity\n- Time complexity:O(2^n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n ans=[]\n def solve(self,array,Sum,candidates,target,start):\n # print([array,Sum])\n if Sum==target:\n self.ans.append(array)\n for index in range(start,len(candidates)):\n i=candidates[index]\n if Sum+i>target:\n # print(Sum+i)\n return\n array1=list(array)\n array1.append(i)\n Sum1=Sum+i\n self.solve(array1,Sum1,candidates,target,index)\n \n \n\n def combinationSum(self, candidates: List[int], target: int) -> List[List[int]]:\n self.ans=[]\n candidates.sort()\n self.solve([],0,candidates,target,0)\n\n return self.ans\n \n \n``` | 2 | Given an array of **distinct** integers `candidates` and a target integer `target`, return _a list of all **unique combinations** of_ `candidates` _where the chosen numbers sum to_ `target`_._ You may return the combinations in **any order**.
The **same** number may be chosen from `candidates` an **unlimited number of times**. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
The test cases are generated such that the number of unique combinations that sum up to `target` is less than `150` combinations for the given input.
**Example 1:**
**Input:** candidates = \[2,3,6,7\], target = 7
**Output:** \[\[2,2,3\],\[7\]\]
**Explanation:**
2 and 3 are candidates, and 2 + 2 + 3 = 7. Note that 2 can be used multiple times.
7 is a candidate, and 7 = 7.
These are the only two combinations.
**Example 2:**
**Input:** candidates = \[2,3,5\], target = 8
**Output:** \[\[2,2,2,2\],\[2,3,3\],\[3,5\]\]
**Example 3:**
**Input:** candidates = \[2\], target = 1
**Output:** \[\]
**Constraints:**
* `1 <= candidates.length <= 30`
* `2 <= candidates[i] <= 40`
* All elements of `candidates` are **distinct**.
* `1 <= target <= 40` | null |
General Backtracking questions solutions in Python for reference : | combination-sum | 0 | 1 | I have taken solutions of @caikehe from frequently asked backtracking questions which I found really helpful and had copied for my reference. I thought this post will be helpful for everybody as in an interview I think these basic solutions can come in handy. Please add any more questions in comments that you think might be important and I can add it in the post.\n\n#### Combinations :\n```\ndef combine(self, n, k):\n res = []\n self.dfs(xrange(1,n+1), k, 0, [], res)\n return res\n \ndef dfs(self, nums, k, index, path, res):\n #if k < 0: #backtracking\n #return \n if k == 0:\n res.append(path)\n return # backtracking \n for i in xrange(index, len(nums)):\n self.dfs(nums, k-1, i+1, path+[nums[i]], res)\n``` \n\t\n#### Permutations I\n```\nclass Solution:\n def permute(self, nums: List[int]) -> List[List[int]]:\n res = []\n self.dfs(nums, [], res)\n return res\n\n def dfs(self, nums, path, res):\n if not nums:\n res.append(path)\n #return # backtracking\n for i in range(len(nums)):\n self.dfs(nums[:i]+nums[i+1:], path+[nums[i]], res)\n``` \n\n#### Permutations II\n```\ndef permuteUnique(self, nums):\n res, visited = [], [False]*len(nums)\n nums.sort()\n self.dfs(nums, visited, [], res)\n return res\n \ndef dfs(self, nums, visited, path, res):\n if len(nums) == len(path):\n res.append(path)\n return \n for i in xrange(len(nums)):\n if not visited[i]: \n if i>0 and not visited[i-1] and nums[i] == nums[i-1]: # here should pay attention\n continue\n visited[i] = True\n self.dfs(nums, visited, path+[nums[i]], res)\n visited[i] = False\n```\n\n \n#### Subsets 1\n\n\n```\ndef subsets1(self, nums):\n res = []\n self.dfs(sorted(nums), 0, [], res)\n return res\n \ndef dfs(self, nums, index, path, res):\n res.append(path)\n for i in xrange(index, len(nums)):\n self.dfs(nums, i+1, path+[nums[i]], res)\n```\n\n\n#### Subsets II \n\n\n```\ndef subsetsWithDup(self, nums):\n res = []\n nums.sort()\n self.dfs(nums, 0, [], res)\n return res\n \ndef dfs(self, nums, index, path, res):\n res.append(path)\n for i in xrange(index, len(nums)):\n if i > index and nums[i] == nums[i-1]:\n continue\n self.dfs(nums, i+1, path+[nums[i]], res)\n```\n\n\n#### Combination Sum \n\n\n```\ndef combinationSum(self, candidates, target):\n res = []\n candidates.sort()\n self.dfs(candidates, target, 0, [], res)\n return res\n \ndef dfs(self, nums, target, index, path, res):\n if target < 0:\n return # backtracking\n if target == 0:\n res.append(path)\n return \n for i in xrange(index, len(nums)):\n self.dfs(nums, target-nums[i], i, path+[nums[i]], res)\n```\n\n \n \n#### Combination Sum II \n\n```\ndef combinationSum2(self, candidates, target):\n res = []\n candidates.sort()\n self.dfs(candidates, target, 0, [], res)\n return res\n \ndef dfs(self, candidates, target, index, path, res):\n if target < 0:\n return # backtracking\n if target == 0:\n res.append(path)\n return # backtracking \n for i in xrange(index, len(candidates)):\n if i > index and candidates[i] == candidates[i-1]:\n continue\n self.dfs(candidates, target-candidates[i], i+1, path+[candidates[i]], res)\n``` | 265 | Given an array of **distinct** integers `candidates` and a target integer `target`, return _a list of all **unique combinations** of_ `candidates` _where the chosen numbers sum to_ `target`_._ You may return the combinations in **any order**.
The **same** number may be chosen from `candidates` an **unlimited number of times**. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
The test cases are generated such that the number of unique combinations that sum up to `target` is less than `150` combinations for the given input.
**Example 1:**
**Input:** candidates = \[2,3,6,7\], target = 7
**Output:** \[\[2,2,3\],\[7\]\]
**Explanation:**
2 and 3 are candidates, and 2 + 2 + 3 = 7. Note that 2 can be used multiple times.
7 is a candidate, and 7 = 7.
These are the only two combinations.
**Example 2:**
**Input:** candidates = \[2,3,5\], target = 8
**Output:** \[\[2,2,2,2\],\[2,3,3\],\[3,5\]\]
**Example 3:**
**Input:** candidates = \[2\], target = 1
**Output:** \[\]
**Constraints:**
* `1 <= candidates.length <= 30`
* `2 <= candidates[i] <= 40`
* All elements of `candidates` are **distinct**.
* `1 <= target <= 40` | null |
Python 3 | DFS/Backtracking & Two DP methods | Explanations | combination-sum | 0 | 1 | ### Approach \\#1. DFS (Backtracking)\n- Straight forward backtracking\n- `cur`: current combination, `cur_sum` current combination sum, `idx` index current at (to avoid repeats)\n- Time complexity: `O(N^(M/min_cand + 1))`, `N = len(candidates)`, `M = target`, `min_cand = min(candidates)`\n- Space complexity: `O(M/min_cand)`\n<iframe src="https://leetcode.com/playground/fwFMybYZ/shared" frameBorder="0" width="850" height="300"></iframe>\n\n### Approach \\#2. DP (Slow)\n- Read comment for more detail\n- Time Complexity: `O(M*M*M*N)`, `N = len(candidates)`, `M = target`\n- Space Complexity: `O(M*M)`\n<iframe src="https://leetcode.com/playground/Qw6fiSbh/shared" frameBorder="0" width="850" height="300"></iframe>\n\n### Approach \\#3. DP (Fast)\n- Read comment for more detail\n- Time Complexity: `O(M*M*N)`, `N = len(candidates)`, `M = target`\n- Space Complexity: `O(M*M)`\n<iframe src="https://leetcode.com/playground/d2qASVHs/shared" frameBorder="0" width="850" height="300"></iframe>\n | 145 | Given an array of **distinct** integers `candidates` and a target integer `target`, return _a list of all **unique combinations** of_ `candidates` _where the chosen numbers sum to_ `target`_._ You may return the combinations in **any order**.
The **same** number may be chosen from `candidates` an **unlimited number of times**. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
The test cases are generated such that the number of unique combinations that sum up to `target` is less than `150` combinations for the given input.
**Example 1:**
**Input:** candidates = \[2,3,6,7\], target = 7
**Output:** \[\[2,2,3\],\[7\]\]
**Explanation:**
2 and 3 are candidates, and 2 + 2 + 3 = 7. Note that 2 can be used multiple times.
7 is a candidate, and 7 = 7.
These are the only two combinations.
**Example 2:**
**Input:** candidates = \[2,3,5\], target = 8
**Output:** \[\[2,2,2,2\],\[2,3,3\],\[3,5\]\]
**Example 3:**
**Input:** candidates = \[2\], target = 1
**Output:** \[\]
**Constraints:**
* `1 <= candidates.length <= 30`
* `2 <= candidates[i] <= 40`
* All elements of `candidates` are **distinct**.
* `1 <= target <= 40` | null |
Backtraking 4 connected problems | combination-sum | 0 | 1 | # 1.combination sum\n```\nclass Solution:\n def combinationSum(self, candidates: List[int], target: int) -> List[List[int]]:\n res=[]\n def dfs(candidates,target,path,res):\n if target==0:\n res.append(path)\n return\n for i in range(len(candidates)):\n if candidates[i]>target:\n continue\n dfs(candidates[i:],target-candidates[i],path+[candidates[i]],res)\n dfs(candidates,target,[],res)\n return res\n```\n# 2.combination sum II\n```\nclass Solution:\n def combinationSum2(self, candidates: List[int], target: int) -> List[List[int]]:\n list1=[]\n candidates.sort()\n def dfs(candidates,target,path,list1):\n if target==0:\n list1.append(path)\n return\n for i in range(len(candidates)):\n if candidates[i]>target:\n continue\n if i>=1 and candidates[i]==candidates[i-1]:\n continue\n dfs(candidates[i+1:],target-candidates[i],path+[candidates[i]],list1)\n dfs(candidates,target,[],list1)\n return list1\n```\n# 3. permutation\n```\nclass Solution:\n def permute(self, nums: List[int]) -> List[List[int]]:\n def back(nums,ans,temp):\n if len(nums)==0:\n ans.append(temp)\n return \n for i in range(len(nums)):\n back(nums[:i]+nums[i+1:],ans,temp+[nums[i]])\n ans=[]\n back(nums,ans,[])\n return ans\n```\n# 4. Restore IP Address\n```\nclass Solution:\n def restoreIpAddresses(self, s: str) -> List[str]:\n ans,k=[],0\n def back(s,ans,k,temp=\'\'):\n if k==4 and len(s)==0:\n ans.append(temp[:-1])\n return\n if k==4 or len(s)==0:\n return \n for i in range(3):\n if k>4 or i+1>len(s):\n break\n if int(s[:i+1])>255:\n continue\n if i!=0 and s[0]=="0":\n continue\n back(s[i+1:],ans,k+1,temp+s[:i+1]+\'.\') \n return ans\n return back(s,ans,k,\'\')\n```\n# please upvote me it would encourage me alot\n\n\n | 12 | Given an array of **distinct** integers `candidates` and a target integer `target`, return _a list of all **unique combinations** of_ `candidates` _where the chosen numbers sum to_ `target`_._ You may return the combinations in **any order**.
The **same** number may be chosen from `candidates` an **unlimited number of times**. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
The test cases are generated such that the number of unique combinations that sum up to `target` is less than `150` combinations for the given input.
**Example 1:**
**Input:** candidates = \[2,3,6,7\], target = 7
**Output:** \[\[2,2,3\],\[7\]\]
**Explanation:**
2 and 3 are candidates, and 2 + 2 + 3 = 7. Note that 2 can be used multiple times.
7 is a candidate, and 7 = 7.
These are the only two combinations.
**Example 2:**
**Input:** candidates = \[2,3,5\], target = 8
**Output:** \[\[2,2,2,2\],\[2,3,3\],\[3,5\]\]
**Example 3:**
**Input:** candidates = \[2\], target = 1
**Output:** \[\]
**Constraints:**
* `1 <= candidates.length <= 30`
* `2 <= candidates[i] <= 40`
* All elements of `candidates` are **distinct**.
* `1 <= target <= 40` | null |
Most optimal solution using recursion and backtracking with complete explanation | combination-sum | 1 | 1 | \n\n# Approach\n- The findAns function is a recursive helper function that takes an index, the remaining target value, the candidates array, the answer vector (ans), and the current helper vector (helper).\n\n- The base case of the recursion is when the current index is equal to the size of the candidates array. If the remaining target value is also 0, it means a valid combination has been found, so the current helper vector is added to the answer vector.\n\n- Within the recursive function, there are two recursive calls:\n - If the current candidate value at the current index is less than or equal to the remaining target value, it means we can include this value in the combination. So, we add the current candidate value to the helper vector, subtract it from the target value, and recursively call findAns with the same index.\n - Regardless of whether the current candidate is included or not, we move on to the next candidate by calling findAns with the next index.\n\n- The combinationSum function initializes the answer vector (ans) and a helper vector (helper), and then calls the findAns function with the initial index (0), the target value, the candidates array, and the empty helper vector.\n\n- Finally, the ans vector containing all valid combinations is returned.\n\n# Complexity\n- Time complexity:\nO((2^t)*k)\n\n- Space complexity:\nO(k*x)\n\n```C++ []\nclass Solution {\npublic:\n void findAns(int index, int target, vector<int>& arr, vector<vector<int>>& ans, vector<int>& helper) {\n if(index == arr.size()) {\n if(target == 0) ans.push_back(helper);\n return;\n }\n if(arr[index] <= target) {\n helper.push_back(arr[index]);\n findAns(index, target-arr[index], arr, ans, helper);\n helper.pop_back();\n }\n findAns(index+1, target, arr, ans, helper);\n }\n vector<vector<int>> combinationSum(vector<int>& candidates, int target) {\n vector<vector<int>> ans;\n vector<int> helper;\n findAns(0, target, candidates, ans, helper);\n return ans;\n }\n};\n```\n```JAVA []\nclass Solution {\n public List<List<Integer>> combinationSum(int[] candidates, int target) {\n List<List<Integer>> ans = new ArrayList<>();\n List<Integer> helper = new ArrayList<>();\n findAns(0, target, candidates, ans, helper);\n return ans;\n }\n \n private void findAns(int index, int target, int[] arr, List<List<Integer>> ans, List<Integer> helper) {\n if (index == arr.length) {\n if (target == 0) ans.add(new ArrayList<>(helper));\n return;\n }\n \n if (arr[index] <= target) {\n helper.add(arr[index]);\n findAns(index, target - arr[index], arr, ans, helper);\n helper.remove(helper.size() - 1);\n }\n \n findAns(index + 1, target, arr, ans, helper);\n }\n}\n\n```\n```Python3 []\nclass Solution:\n def combinationSum(self, candidates: List[int], target: int) -> List[List[int]]:\n ans = []\n helper = []\n self.findAns(0, target, candidates, ans, helper)\n return ans\n \n def findAns(self, index, target, arr, ans, helper):\n if index == len(arr):\n if target == 0:\n ans.append(helper[:])\n return\n \n if arr[index] <= target:\n helper.append(arr[index])\n self.findAns(index, target - arr[index], arr, ans, helper)\n helper.pop()\n \n self.findAns(index + 1, target, arr, ans, helper)\n\n```\n\n``` | 4 | Given an array of **distinct** integers `candidates` and a target integer `target`, return _a list of all **unique combinations** of_ `candidates` _where the chosen numbers sum to_ `target`_._ You may return the combinations in **any order**.
The **same** number may be chosen from `candidates` an **unlimited number of times**. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
The test cases are generated such that the number of unique combinations that sum up to `target` is less than `150` combinations for the given input.
**Example 1:**
**Input:** candidates = \[2,3,6,7\], target = 7
**Output:** \[\[2,2,3\],\[7\]\]
**Explanation:**
2 and 3 are candidates, and 2 + 2 + 3 = 7. Note that 2 can be used multiple times.
7 is a candidate, and 7 = 7.
These are the only two combinations.
**Example 2:**
**Input:** candidates = \[2,3,5\], target = 8
**Output:** \[\[2,2,2,2\],\[2,3,3\],\[3,5\]\]
**Example 3:**
**Input:** candidates = \[2\], target = 1
**Output:** \[\]
**Constraints:**
* `1 <= candidates.length <= 30`
* `2 <= candidates[i] <= 40`
* All elements of `candidates` are **distinct**.
* `1 <= target <= 40` | null |
Backtracking with Python3 well Explantation (Easy & Clear) | combination-sum | 0 | 1 | # Intuition:\nThe problem requires finding all possible combinations of elements from a given list of integers such that their sum is equal to a target value. The approach is to use backtracking to generate all possible combinations and keep track of the valid ones.\n\n# Approach:\nThe given code defines a class Solution and a function combinationSum that takes a list of integers and a target value as input and returns a list of lists containing all valid combinations of integers that add up to the target value.\n\nThe approach used is recursive backtracking. The backtracking function takes two arguments, curr which represents the current combination and i which represents the index of the next element to be added to the combination.\n\nInitially, the current combination is empty and the index i is set to zero. Then, for each index j starting from i, the backtracking function is called recursively with the updated combination curr+[can[j]] and the index j. This ensures that all possible combinations are explored.\n\nThe function also checks if the current combination sum equals the target value and adds it to the result list if it is valid.\n\n# Complexity:\n# Time complexity: \nThe time complexity of the backtracking function is O(2^n), where n is the length of the input list can. This is because there are 2^n possible combinations of elements from the input list. Therefore, the time complexity of the entire function is O(2^n) as well.\n\n# Space complexity: \nThe space complexity of the backtracking function is O(n) as the maximum number of elements that can be stored in the current combination at any point is n. The space complexity of the entire function is also O(2^n) as there can be at most 2^n valid combinations.\n\n# Code\n```\nclass Solution:\n def combinationSum(self, can: List[int], target: int) -> List[List[int]]:\n res=[]\n def backtracking(curr: List[int],i:int):\n s=sum(curr)\n if s==target:\n res.append(curr)\n elif s<target:\n for j in range(i,len(can)):\n backtracking(curr+[can[j]],j)\n backtracking([],0)\n return res\n``` | 11 | Given an array of **distinct** integers `candidates` and a target integer `target`, return _a list of all **unique combinations** of_ `candidates` _where the chosen numbers sum to_ `target`_._ You may return the combinations in **any order**.
The **same** number may be chosen from `candidates` an **unlimited number of times**. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
The test cases are generated such that the number of unique combinations that sum up to `target` is less than `150` combinations for the given input.
**Example 1:**
**Input:** candidates = \[2,3,6,7\], target = 7
**Output:** \[\[2,2,3\],\[7\]\]
**Explanation:**
2 and 3 are candidates, and 2 + 2 + 3 = 7. Note that 2 can be used multiple times.
7 is a candidate, and 7 = 7.
These are the only two combinations.
**Example 2:**
**Input:** candidates = \[2,3,5\], target = 8
**Output:** \[\[2,2,2,2\],\[2,3,3\],\[3,5\]\]
**Example 3:**
**Input:** candidates = \[2\], target = 1
**Output:** \[\]
**Constraints:**
* `1 <= candidates.length <= 30`
* `2 <= candidates[i] <= 40`
* All elements of `candidates` are **distinct**.
* `1 <= target <= 40` | null |
Python3 Recursive Solution | combination-sum | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def combinationSum(self, candidates: List[int], target: int) -> List[List[int]]:\n ans=[]\n n=len(candidates)\n def solve(idx,sum,lst):\n if idx>=n:\n if sum==target:\n ans.append(lst)\n return \n solve(idx+1,sum,lst)\n if sum+candidates[idx]<=target:\n solve(idx,sum+candidates[idx],lst+[candidates[idx]])\n solve(0,0,[])\n return ans\n``` | 1 | Given an array of **distinct** integers `candidates` and a target integer `target`, return _a list of all **unique combinations** of_ `candidates` _where the chosen numbers sum to_ `target`_._ You may return the combinations in **any order**.
The **same** number may be chosen from `candidates` an **unlimited number of times**. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
The test cases are generated such that the number of unique combinations that sum up to `target` is less than `150` combinations for the given input.
**Example 1:**
**Input:** candidates = \[2,3,6,7\], target = 7
**Output:** \[\[2,2,3\],\[7\]\]
**Explanation:**
2 and 3 are candidates, and 2 + 2 + 3 = 7. Note that 2 can be used multiple times.
7 is a candidate, and 7 = 7.
These are the only two combinations.
**Example 2:**
**Input:** candidates = \[2,3,5\], target = 8
**Output:** \[\[2,2,2,2\],\[2,3,3\],\[3,5\]\]
**Example 3:**
**Input:** candidates = \[2\], target = 1
**Output:** \[\]
**Constraints:**
* `1 <= candidates.length <= 30`
* `2 <= candidates[i] <= 40`
* All elements of `candidates` are **distinct**.
* `1 <= target <= 40` | null |
Python || BackTracking || Easy TO Understand 🔥 | combination-sum | 0 | 1 | # Code\n```\nclass Solution:\n def combinationSum(self, candidates: List[int], target: int) -> List[List[int]]:\n def comb(i, target, ds, ans):\n if i == len(candidates):\n if target == 0 and ds not in ans:\n ans.append(ds.copy())\n return\n if candidates[i] <= target:\n ds.append(candidates[i])\n comb(i, target - candidates[i], ds, ans)\n ds.pop()\n comb(i + 1, target, ds, ans)\n return ans\n return comb(0, target, ds = [], ans = [])\n``` | 2 | Given an array of **distinct** integers `candidates` and a target integer `target`, return _a list of all **unique combinations** of_ `candidates` _where the chosen numbers sum to_ `target`_._ You may return the combinations in **any order**.
The **same** number may be chosen from `candidates` an **unlimited number of times**. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
The test cases are generated such that the number of unique combinations that sum up to `target` is less than `150` combinations for the given input.
**Example 1:**
**Input:** candidates = \[2,3,6,7\], target = 7
**Output:** \[\[2,2,3\],\[7\]\]
**Explanation:**
2 and 3 are candidates, and 2 + 2 + 3 = 7. Note that 2 can be used multiple times.
7 is a candidate, and 7 = 7.
These are the only two combinations.
**Example 2:**
**Input:** candidates = \[2,3,5\], target = 8
**Output:** \[\[2,2,2,2\],\[2,3,3\],\[3,5\]\]
**Example 3:**
**Input:** candidates = \[2\], target = 1
**Output:** \[\]
**Constraints:**
* `1 <= candidates.length <= 30`
* `2 <= candidates[i] <= 40`
* All elements of `candidates` are **distinct**.
* `1 <= target <= 40` | null |
✅ Python short and clean solution explained | combination-sum | 0 | 1 | ```\nclass Solution:\n def combinationSum(self, candidates: List[int], target: int) -> List[List[int]]:\n self.ans = [] # for adding all the answers\n def traverse(candid, arr,sm): # arr : an array that contains the accused combination; sm : is the sum of all elements of arr \n if sm == target: self.ans.append(arr) # If sum is equal to target then you know it, I know it, what to do\n if sm >= target: return # If sum is greater than target then no need to move further.\n for i in range(len(candid)): # we will traverse each element from the array.\n traverse(candid[i:], arr + [candid[i]], sm+candid[i]) #most important, splice the array including the current index, splicing in order to handle the duplicates.\n traverse(candidates,[], 0)\n return self.ans\n``` | 40 | Given an array of **distinct** integers `candidates` and a target integer `target`, return _a list of all **unique combinations** of_ `candidates` _where the chosen numbers sum to_ `target`_._ You may return the combinations in **any order**.
The **same** number may be chosen from `candidates` an **unlimited number of times**. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
The test cases are generated such that the number of unique combinations that sum up to `target` is less than `150` combinations for the given input.
**Example 1:**
**Input:** candidates = \[2,3,6,7\], target = 7
**Output:** \[\[2,2,3\],\[7\]\]
**Explanation:**
2 and 3 are candidates, and 2 + 2 + 3 = 7. Note that 2 can be used multiple times.
7 is a candidate, and 7 = 7.
These are the only two combinations.
**Example 2:**
**Input:** candidates = \[2,3,5\], target = 8
**Output:** \[\[2,2,2,2\],\[2,3,3\],\[3,5\]\]
**Example 3:**
**Input:** candidates = \[2\], target = 1
**Output:** \[\]
**Constraints:**
* `1 <= candidates.length <= 30`
* `2 <= candidates[i] <= 40`
* All elements of `candidates` are **distinct**.
* `1 <= target <= 40` | null |
Easy to understand python recursion!!! | combination-sum | 0 | 1 | \n# Code\n```\nclass Solution:\n def combinationSum(self, candidates: List[int], target: int) -> List[List[int]]:\n res = []\n\n def solve(cand, t, pre, arr):\n if pre == t:\n res.append(sorted(arr.copy()))\n \n if pre > t:\n return\n \n for i in cand:\n arr.append(i)\n solve(cand, t, pre + i, arr)\n arr.pop()\n \n solve(candidates, target, 0, [])\n \n n = len(res)\n ans = []\n for i in range(n):\n if res[i] in ans:\n continue\n ans.append(res[i])\n return ans\n``` | 2 | Given an array of **distinct** integers `candidates` and a target integer `target`, return _a list of all **unique combinations** of_ `candidates` _where the chosen numbers sum to_ `target`_._ You may return the combinations in **any order**.
The **same** number may be chosen from `candidates` an **unlimited number of times**. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
The test cases are generated such that the number of unique combinations that sum up to `target` is less than `150` combinations for the given input.
**Example 1:**
**Input:** candidates = \[2,3,6,7\], target = 7
**Output:** \[\[2,2,3\],\[7\]\]
**Explanation:**
2 and 3 are candidates, and 2 + 2 + 3 = 7. Note that 2 can be used multiple times.
7 is a candidate, and 7 = 7.
These are the only two combinations.
**Example 2:**
**Input:** candidates = \[2,3,5\], target = 8
**Output:** \[\[2,2,2,2\],\[2,3,3\],\[3,5\]\]
**Example 3:**
**Input:** candidates = \[2\], target = 1
**Output:** \[\]
**Constraints:**
* `1 <= candidates.length <= 30`
* `2 <= candidates[i] <= 40`
* All elements of `candidates` are **distinct**.
* `1 <= target <= 40` | null |
🚀 [VIDEO] Mastering A Journey into Recursion & DFS | combination-sum | 0 | 1 | # Intuition\nFor this problem, the initial thoughts revolve around the concept of recursive computation. Since we can choose a number multiple times, and we need to find all possible combinations, we can start by choosing a number, subtract it from the target, and then recursively repeat this process. If we hit the target exactly, we\'ve found a valid combination. If we overshoot, we can discard this combination.\n\nhttps://youtu.be/CWbkawwtwvU\n\n# Approach\nThe approach involves a recursive function, often termed as depth-first search (DFS) in this context. We recursively choose each number in the input list and subtract it from the target until we either hit the target (in which case we\'ve found a valid combination), or overshoot it (in which case we discard the combination). We do this recursively for all the numbers, and each time we hit the target, we add the combination to our result.\n\n# Complexity\n- Time complexity: The time complexity of this solution is $$O(n^k)$$, where n is the number of candidates and k is the maximum repetitions of a single number in the combination. This is because in the worst case, we have to explore each possible combination. \n\n- Space complexity: The space complexity is $$O(k)$$, as in the process of finding combinations, we store a combination in memory. k is the maximum length of a combination.\n\n# Code\n``` Python []\nclass Solution:\n def combinationSum(self, candidates: List[int], target: int) -> List[List[int]]:\n result = [] \n self.dfs(candidates, target, [], result) \n return result \n\n def dfs(self, nums, target, path, result): \n if target < 0: \n # backtracking \n return \n if target == 0: \n result.append(path) \n return \n for i in range(len(nums)): \n self.dfs(nums[i:], target-nums[i], path+[nums[i]], result) \n```\n``` JavaScript []\nvar combinationSum = function(candidates, target) {\n let result = []; \n dfs(candidates, target, [], result); \n return result; \n \n};\n\nfunction dfs(nums, target, path, result) { \n if(target < 0){ \n // Backtracking \n return; \n } \n if(target === 0){ \n result.push(path); \n return; \n } \n for(let i = 0; i < nums.length; i++){ \n dfs(nums.slice(i), target - nums[i], [...path, nums[i]], result); \n } \n} \n \n``` | 3 | Given an array of **distinct** integers `candidates` and a target integer `target`, return _a list of all **unique combinations** of_ `candidates` _where the chosen numbers sum to_ `target`_._ You may return the combinations in **any order**.
The **same** number may be chosen from `candidates` an **unlimited number of times**. Two combinations are unique if the frequency of at least one of the chosen numbers is different.
The test cases are generated such that the number of unique combinations that sum up to `target` is less than `150` combinations for the given input.
**Example 1:**
**Input:** candidates = \[2,3,6,7\], target = 7
**Output:** \[\[2,2,3\],\[7\]\]
**Explanation:**
2 and 3 are candidates, and 2 + 2 + 3 = 7. Note that 2 can be used multiple times.
7 is a candidate, and 7 = 7.
These are the only two combinations.
**Example 2:**
**Input:** candidates = \[2,3,5\], target = 8
**Output:** \[\[2,2,2,2\],\[2,3,3\],\[3,5\]\]
**Example 3:**
**Input:** candidates = \[2\], target = 1
**Output:** \[\]
**Constraints:**
* `1 <= candidates.length <= 30`
* `2 <= candidates[i] <= 40`
* All elements of `candidates` are **distinct**.
* `1 <= target <= 40` | null |
Bad Code! - Recursion in Py | combination-sum-ii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nSort the array and try out all possible subsequence, thinking in terms of whatif my subsequence starts with this particular element. How it will progress? till where it go?\n# Approach\n<!-- Describe your approach to solving the problem. -->\nSimiliar to combination sum variant 1 \n# Complexity\n- Time complexity: k*2^n\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n*k), where k is length of combination\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def comb(self, array, idx, ans, out, target):\n if target == 0:\n ans.append(out.copy())\n return\n if idx == len(array):\n return\n for i in range(idx,len(array)):\n if array[i] > target:\n break\n if i!=idx and array[i] == array[i-1]:\n continue\n out.append(array[i])\n self.comb(array, i+1, ans, out, target-array[i])\n out.pop(-1)\n \n\n def combinationSum2(self, candidates: List[int], target: int) -> List[List[int]]:\n candidates.sort()\n ans = []\n self.comb(candidates, 0, ans, [], target)\n return ans\n \n``` | 0 | Given a collection of candidate numbers (`candidates`) and a target number (`target`), find all unique combinations in `candidates` where the candidate numbers sum to `target`.
Each number in `candidates` may only be used **once** in the combination.
**Note:** The solution set must not contain duplicate combinations.
**Example 1:**
**Input:** candidates = \[10,1,2,7,6,1,5\], target = 8
**Output:**
\[
\[1,1,6\],
\[1,2,5\],
\[1,7\],
\[2,6\]
\]
**Example 2:**
**Input:** candidates = \[2,5,2,1,2\], target = 5
**Output:**
\[
\[1,2,2\],
\[5\]
\]
**Constraints:**
* `1 <= candidates.length <= 100`
* `1 <= candidates[i] <= 50`
* `1 <= target <= 30` | null |
Easy Explanation using Images and Dry run - Using Set & Without Set🐍👍 | combination-sum-ii | 1 | 1 | # Understanding the Question\nHELLO! Lets analyze how to solve this problem. well if you are solving this problem then i am assuming that you already solved **Combination Sum 1 problem** , Where we could pick any value multiple times. \nThis problem is a little different. Here we cant choose a single value multiple times, also here is one more thing. if we get ans=[[1,7],[7,1]] then our final ans will be [[1,7]] as duplicate combinations are not allowed.\n\n# Approach\nIn combination sum 1 problem we had two choices--\n- Either pick the current element and again pick the same element.\n**OR**\n- dont pick the current element, move 1 step ahead and repeat the same process\n\nBut in combination sum 2 problem we cant choose one value multiple times. so what options do we have?\n- Either choose the value and move ahead\n**OR**\n- Dont pick the value and move ahead\n\nso how will our recurrence relations will look?\n\n- f(i+1,sum-arr[i],arr) ***Pick the value and move on to next index***\n**OR**\n- f(i+1,sum,arr) ***dont pick the value but move onto next index***\n\nnow take a simple example to understand recursive calls:\nlets take **arr=[1,1,7]** and **target=8**\nhere i have attached a picture where pick and not_pick calls happens\n*(pardon my drawing, i draw using trackpad)*\n\n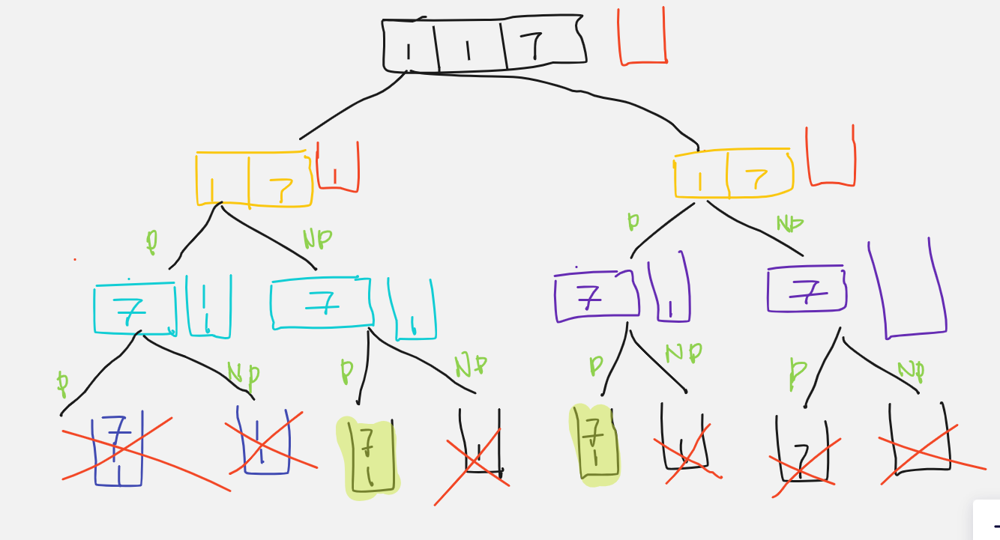\n\nhere as you can see at last we are getting 2 answers [[1,7],[1,7]]\nbut as we know duplicate combinations are not allowed so we have to find a way to only get one set of combination.\n\n***There are Two ways-***\n1. Using Set Data Structure\n2. Using Brain\n\n*i will not talk about how to use set as it is very easy.Although i will share the code using set ds.*\n\n**Lets talk about the second approach--**\n1. if you carefully observe the answer of test cases in this problem you can notice that all the combination set are sorted. means [1,7] then [2,6] this way.\nso to achive combinations in sorted order we need to sort our arr as well.\n2. our main reason why we are getting [1,7] twice is because there is two 1 in our arr.\nif u see previous image which i attached u will see that when i picked the 1 **[0th index]** i got [1,7] as an answer combination. \nand when i **didn\'t picked** the 1 **[0th index]** i had another 1 **[1st index]** which is going in the next recursive call and giving me an extra [1,7] combination.\n\n3. so if i can manipulate my code in a way that at the time of not picking the element, which i already picked **1** **[0th index]** ,will not go to the not pick call with the same value then we can avoid getting the duplicate.\n\n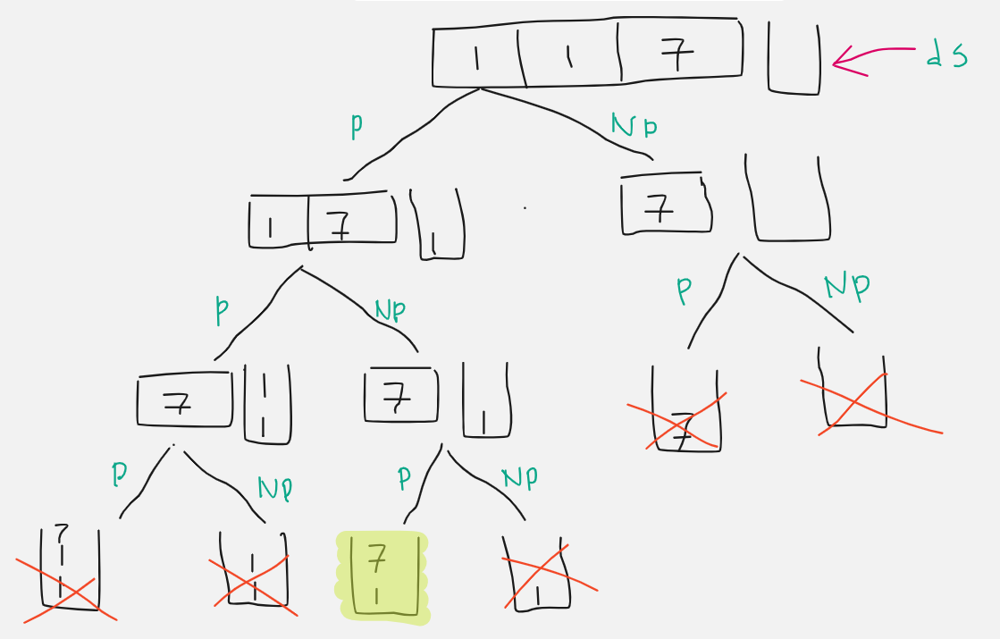\n\n4. see here i picked 1**[0th index]** which is okay.\n5. but when i am not picking 1[0th index], i am not letting any 1 value pass to the not pick call.\n6. i have picked 1 so at the time of not pick i\'ll avoid all the 1 present in the array.\n7. to skip all the 1, i have used a loop. \n8. pick 1 and go to next index. \nbut if dont pick 1 then dont pick any 1 value and go directly to the next unique element which is 7.\n\nI am not explaning about the base case here. if you r having hard time finding how i got the base case then plz comment down below. I will reply.\n\n***now see the above 2 picture again. spot the difference.***\n# PLZ UPVOTE IF YOU UNDERSTOOD THE APPROACH (\u25CF\'\u25E1\'\u25CF)\n\n# Time Complexity:\nTime complexity will be $O(2^n*n)$\n$2^n$ because every element have two choices either pick or not pick.\nand n extra because we are using a while loop inside the recursive function which will add n time complexity.\n\n# Code using SET\n```\nclass Solution:\n def f(self,i,arr,target,ds,ans):\n # BASE CASE\n if i==len(arr):\n if target==0:\n ans.add(tuple(ds))\n return\n\n # RECURENCE RELATION\n if target>=arr[i]:\n ds.append(arr[i])\n self.f(i+1,arr,target-arr[i],ds,ans)\n ds.pop()\n self.f(i+1,arr,target,ds,ans)\n\n def combinationSum2(self, candidates: List[int], target: int) -> List[List[int]]:\n ds=[]\n ans=set()\n candidates.sort()\n self.f(0,candidates,target,ds,ans)\n return [list(combination) for combination in ans]\n```\n\n# Code Using Brain\n```\nclass Solution:\n def f(self,i,arr,target,ds,ans):\n # BASE CASE\n if target==0:\n ans.append(ds.copy())\n return\n if i>=len(arr):\n return\n # RECURENCE RELATION\n if target>=arr[i]:\n ds.append(arr[i])\n self.f(i+1,arr,target-arr[i],ds,ans) #PICK\n ds.pop()\n j=i+1\n while(j<len(arr) and arr[j]==arr[j-1]):\n j+=1\n self.f(j,arr,target,ds,ans) #NOT PICK\n\n def combinationSum2(self, candidates: List[int], target: int) -> List[List[int]]:\n ds=[]\n ans=[]\n candidates.sort()\n self.f(0,candidates,target,ds,ans)\n return ans\n``` | 17 | Given a collection of candidate numbers (`candidates`) and a target number (`target`), find all unique combinations in `candidates` where the candidate numbers sum to `target`.
Each number in `candidates` may only be used **once** in the combination.
**Note:** The solution set must not contain duplicate combinations.
**Example 1:**
**Input:** candidates = \[10,1,2,7,6,1,5\], target = 8
**Output:**
\[
\[1,1,6\],
\[1,2,5\],
\[1,7\],
\[2,6\]
\]
**Example 2:**
**Input:** candidates = \[2,5,2,1,2\], target = 5
**Output:**
\[
\[1,2,2\],
\[5\]
\]
**Constraints:**
* `1 <= candidates.length <= 100`
* `1 <= candidates[i] <= 50`
* `1 <= target <= 30` | null |
[Python3] DFS solutions/templates to 6 different classic backtracking problems & more | combination-sum-ii | 0 | 1 | I have compiled solutions for all the 6 classic backtracking problems, you can practise them together for better understanding. Good luck with your preparation/interviews! \n\n[39. Combination Sum](https://leetcode.com/problems/combination-sum/)\n```\nclass Solution:\n def combinationSum(self, candidates: List[int], target: int) -> List[List[int]]:\n if not candidates: return []\n res = []\n candidates.sort()\n def dfs(idx, path, cur):\n if cur > target: return\n if cur == target:\n res.append(path)\n return\n for i in range(idx, len(candidates)):\n dfs(i, path+[candidates[i]], cur+candidates[i])\n dfs(0, [], 0)\n return res\n```\n\n[40. Combination Sum II](https://leetcode.com/problems/combination-sum-ii/discuss/?currentPage=1&orderBy=recent_activity&query=)\n```\nclass Solution:\n def combinationSum2(self, candidates: List[int], target: int) -> List[List[int]]:\n res = []\n candidates.sort()\n def dfs(idx, path, cur):\n if cur > target: return\n if cur == target:\n res.append(path)\n return\n for i in range(idx, len(candidates)):\n if i > idx and candidates[i] == candidates[i-1]:\n continue\n dfs(i+1, path+[candidates[i]], cur+candidates[i])\n dfs(0, [], 0)\n return res\n```\n[78. Subsets](https://leetcode.com/problems/subsets/)\n```\nclass Solution:\n def subsets(self, nums: List[int]) -> List[List[int]]:\n res = []\n def dfs(idx, path):\n res.append(path)\n for i in range(idx, len(nums)):\n dfs(i+1, path+[nums[i]])\n dfs(0, [])\n return res\n```\n\n[90. Subsets II](https://leetcode.com/problems/subsets-ii/)\n```\nclass Solution:\n def subsetsWithDup(self, nums: List[int]) -> List[List[int]]:\n res = []\n nums.sort()\n def dfs(idx, path):\n res.append(path)\n for i in range(idx, len(nums)):\n if i > idx and nums[i] == nums[i-1]:\n continue\n dfs(i+1, path+[nums[i]])\n dfs(0, [])\n return res\n```\n\n[46. Permutations](https://leetcode.com/problems/permutations/)\n```\nclass Solution:\n def permute(self, nums: List[int]) -> List[List[int]]:\n res = []\n def dfs(counter, path):\n if len(path) == len(nums):\n res.append(path)\n return\n for x in counter:\n if counter[x]:\n counter[x] -= 1\n dfs(counter, path+[x])\n counter[x] += 1\n dfs(Counter(nums), [])\n return res \n```\n\n[47. Permutations II](https://leetcode.com/problems/permutations-ii/)\n```\nclass Solution:\n def permuteUnique(self, nums: List[int]) -> List[List[int]]:\n res = []\n def dfs(counter, path):\n if len(path) == len(nums):\n res.append(path)\n return\n for x in counter:\n if counter[x]:\n counter[x] -= 1\n dfs(counter, path+[x])\n counter[x] += 1\n dfs(Counter(nums), [])\n return res\n```\n\nMore good backtracking problems for practice:\n[131. Palindrome Partitioning](https://leetcode.com/problems/palindrome-partitioning/)\n[784. Lettercase Permutation](https://leetcode.com/problems/letter-case-permutation/)\n[1087. Brace Expansion](https://leetcode.com/problems/brace-expansion/)\n[93. Restore IP addresses](https://leetcode.com/problems/restore-ip-addresses/)\n[1079 Letter Tile Possibilities](https://leetcode.com/problems/letter-tile-possibilities/) | 149 | Given a collection of candidate numbers (`candidates`) and a target number (`target`), find all unique combinations in `candidates` where the candidate numbers sum to `target`.
Each number in `candidates` may only be used **once** in the combination.
**Note:** The solution set must not contain duplicate combinations.
**Example 1:**
**Input:** candidates = \[10,1,2,7,6,1,5\], target = 8
**Output:**
\[
\[1,1,6\],
\[1,2,5\],
\[1,7\],
\[2,6\]
\]
**Example 2:**
**Input:** candidates = \[2,5,2,1,2\], target = 5
**Output:**
\[
\[1,2,2\],
\[5\]
\]
**Constraints:**
* `1 <= candidates.length <= 100`
* `1 <= candidates[i] <= 50`
* `1 <= target <= 30` | null |
Backtracking concept | combination-sum-ii | 0 | 1 | # Backtracking Logic\n```\nclass Solution:\n def combinationSum2(self, candidates: List[int], target: int) -> List[List[int]]:\n list1=[]\n candidates.sort()\n def dfs(candidates,target,path,list1):\n if target==0:\n list1.append(path)\n return\n for i in range(len(candidates)):\n if candidates[i]>target:\n continue\n if i>=1 and candidates[i]==candidates[i-1]:\n continue\n dfs(candidates[i+1:],target-candidates[i],path+[candidates[i]],list1)\n dfs(candidates,target,[],list1)\n return list1\n```\n# please upvote me it would encourage me alot\n | 7 | Given a collection of candidate numbers (`candidates`) and a target number (`target`), find all unique combinations in `candidates` where the candidate numbers sum to `target`.
Each number in `candidates` may only be used **once** in the combination.
**Note:** The solution set must not contain duplicate combinations.
**Example 1:**
**Input:** candidates = \[10,1,2,7,6,1,5\], target = 8
**Output:**
\[
\[1,1,6\],
\[1,2,5\],
\[1,7\],
\[2,6\]
\]
**Example 2:**
**Input:** candidates = \[2,5,2,1,2\], target = 5
**Output:**
\[
\[1,2,2\],
\[5\]
\]
**Constraints:**
* `1 <= candidates.length <= 100`
* `1 <= candidates[i] <= 50`
* `1 <= target <= 30` | null |
✅ Clean and Easy solution - backtracking based Python | combination-sum-ii | 0 | 1 | # Intuition\nThe problem asks for all unique combinations of numbers in the candidates list that sum up to the target. Since each number can only be used once and we want unique combinations, **sorting** the candidates array can be advantageous. \n\nIt allows us to easily `skip over duplicate elements and make the summing up process more straightforward.`\n\n# Approach\n1. Sort the candidates array.\n2. Use backtracking to explore all possible combinations:\n3. If the current sum matches the target, append the current combination to the result.\n4. If the current sum exceeds the target or if all candidates are exhausted, terminate the current branch.\n5. To avoid duplicate combinations, skip over consecutive identical candidates when backtracking.\n\n# Complexity\n**Time complexity:**\nO(2^n) - In the worst case, we might explore all subsets.\n\n**Space complexity:**\nO(n) - Space required for the output list and the recursion stack.\n\n# Code\n```\nclass Solution:\n def combinationSum2(self, candidates: List[int], target: int) -> List[List[int]]:\n candidates.sort()\n output = []\n stack = []\n\n def backtrack(i, total=0):\n if total == target:\n output.append(stack.copy())\n return\n\n if i >= len(candidates) or total > target:\n return\n \n stack.append(candidates[i])\n backtrack(i+1, total+candidates[i])\n\n stack.pop()\n while i+1 < len(candidates) and candidates[i] == candidates[i+1]:\n i += 1\n \n backtrack(i+1, total)\n \n backtrack(0)\n return output\n\n``` | 2 | Given a collection of candidate numbers (`candidates`) and a target number (`target`), find all unique combinations in `candidates` where the candidate numbers sum to `target`.
Each number in `candidates` may only be used **once** in the combination.
**Note:** The solution set must not contain duplicate combinations.
**Example 1:**
**Input:** candidates = \[10,1,2,7,6,1,5\], target = 8
**Output:**
\[
\[1,1,6\],
\[1,2,5\],
\[1,7\],
\[2,6\]
\]
**Example 2:**
**Input:** candidates = \[2,5,2,1,2\], target = 5
**Output:**
\[
\[1,2,2\],
\[5\]
\]
**Constraints:**
* `1 <= candidates.length <= 100`
* `1 <= candidates[i] <= 50`
* `1 <= target <= 30` | null |
Python3, Backtracking, mega easy to understand | combination-sum-ii | 0 | 1 | # Code\n```\nclass Solution:\n def combinationSum2(self, candidates: List[int], target: int) -> List[List[int]]:\n ans = []\n candidates.sort()\n\n def backtracking(start, total, path):\n if total == target:\n ans.append(path)\n return\n \n for i in range(start, len(candidates)):\n if total + candidates[i] > target:\n return\n elif i > start and candidates[i] == candidates[i-1]:\n continue\n else:\n backtracking(i + 1, total + candidates[i], path + [candidates[i]])\n\n backtracking(0, 0, [])\n return ans\n``` | 3 | Given a collection of candidate numbers (`candidates`) and a target number (`target`), find all unique combinations in `candidates` where the candidate numbers sum to `target`.
Each number in `candidates` may only be used **once** in the combination.
**Note:** The solution set must not contain duplicate combinations.
**Example 1:**
**Input:** candidates = \[10,1,2,7,6,1,5\], target = 8
**Output:**
\[
\[1,1,6\],
\[1,2,5\],
\[1,7\],
\[2,6\]
\]
**Example 2:**
**Input:** candidates = \[2,5,2,1,2\], target = 5
**Output:**
\[
\[1,2,2\],
\[5\]
\]
**Constraints:**
* `1 <= candidates.length <= 100`
* `1 <= candidates[i] <= 50`
* `1 <= target <= 30` | null |
Most optimal solution using advanced backtracking and recursion | Beats 100% | Solution Explained | combination-sum-ii | 1 | 1 | \n# Approach\n- The `findAns` function is a recursive helper function that takes an index, the remaining target value, the candidates array, the answer vector (`ans`), and the current helper vector (`helper`).\n\n- The base case of the recursion is when the target value becomes 0. This means a valid combination has been found, so the current helper vector is added to the answer vector.\n\n- Within the recursive function, a loop iterates through the candidates starting from the current index. For each candidate, the following conditions are checked:\n - If the current candidate is the same as the previous candidate, it\'s skipped to avoid duplicate combinations.\n - If the current candidate is greater than the remaining target, it\'s not feasible to include it, so the loop breaks.\n - Otherwise, the current candidate is included in the helper vector, and the recursive call is made with the next index and the updated target value.\n\n- After the loop, the current candidate is removed from the helper vector (backtracking).\n\n- The `combinationSum2` function first sorts the candidates array. This sorting helps in efficiently avoiding duplicate combinations and breaking out of the loop early when candidates exceed the target.\n\n- The `ans`vector containing all valid unique combinations is returned.\n\n# Complexity\n- Time complexity:\nO((2^n)*k)\n\n- Space complexity:\nO(k*x)\n\n```C++ []\nclass Solution {\npublic:\n void findAns(int index, int target, vector<int>& arr, vector<vector<int>>& ans, vector<int>& helper) {\n if(target == 0) {\n ans.push_back(helper);\n return;\n }\n for(int i = index; i < arr.size(); i++) {\n if(i > index && arr[i] ==arr[i-1]) continue;\n if(arr[i] > target) break;\n helper.push_back(arr[i]);\n findAns(i+1, target-arr[i], arr, ans, helper);\n helper.pop_back();\n }\n }\n vector<vector<int>> combinationSum2(vector<int>& candidates, int target) {\n sort(candidates.begin(), candidates.end());\n vector<vector<int>> ans;\n vector<int> helper;\n findAns(0, target, candidates, ans, helper);\n return ans;\n }\n};\n```\n```JAVA []\nclass Solution {\n public List<List<Integer>> combinationSum2(int[] candidates, int target) {\n Arrays.sort(candidates);\n List<List<Integer>> ans = new ArrayList<>();\n List<Integer> helper = new ArrayList<>();\n findAns(0, target, candidates, ans, helper);\n return ans;\n }\n \n private void findAns(int index, int target, int[] arr, List<List<Integer>> ans, List<Integer> helper) {\n if (target == 0) {\n ans.add(new ArrayList<>(helper));\n return;\n }\n \n for (int i = index; i < arr.length; i++) {\n if (i > index && arr[i] == arr[i - 1]) {\n continue;\n }\n if (arr[i] > target) {\n break;\n }\n helper.add(arr[i]);\n findAns(i + 1, target - arr[i], arr, ans, helper);\n helper.remove(helper.size() - 1);\n }\n }\n}\n\n```\n```Python []\nclass Solution:\n def combinationSum2(self, candidates: List[int], target: int) -> List[List[int]]:\n candidates.sort()\n ans = []\n helper = []\n self.findAns(0, target, candidates, ans, helper)\n return ans\n \n def findAns(self, index, target, arr, ans, helper):\n if target == 0:\n ans.append(helper[:])\n return\n \n for i in range(index, len(arr)):\n if i > index and arr[i] == arr[i - 1]:\n continue\n if arr[i] > target:\n break\n helper.append(arr[i])\n self.findAns(i + 1, target - arr[i], arr, ans, helper)\n helper.pop()\n\n```\n | 7 | Given a collection of candidate numbers (`candidates`) and a target number (`target`), find all unique combinations in `candidates` where the candidate numbers sum to `target`.
Each number in `candidates` may only be used **once** in the combination.
**Note:** The solution set must not contain duplicate combinations.
**Example 1:**
**Input:** candidates = \[10,1,2,7,6,1,5\], target = 8
**Output:**
\[
\[1,1,6\],
\[1,2,5\],
\[1,7\],
\[2,6\]
\]
**Example 2:**
**Input:** candidates = \[2,5,2,1,2\], target = 5
**Output:**
\[
\[1,2,2\],
\[5\]
\]
**Constraints:**
* `1 <= candidates.length <= 100`
* `1 <= candidates[i] <= 50`
* `1 <= target <= 30` | null |
Runtime (Beats 97.33%) Combination Sum II with step by step explanation | combination-sum-ii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nSort the candidates list in ascending order.\nDefine a backtracking function that takes in 3 parameters: start index, a list path to store each combination, and the target value.\nIf the target value is 0, append the path list to the result res list.\nLoop through the candidates list starting from the given start index.\nIf the current index i is greater than start and the current candidate is the same as the previous candidate, continue to the next iteration.\nIf the current candidate is greater than the target value, break the loop.\nAdd the current candidate to the path list and call the backtracking function with i + 1 as the start index, the updated path list, and the target minus the current candidate.\nReturn the result res list after the backtracking function is finished.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def combinationSum2(self, candidates: List[int], target: int) -> List[List[int]]:\n def backtrack(start, path, target):\n if target == 0:\n res.append(path)\n return\n for i in range(start, len(candidates)):\n if i > start and candidates[i] == candidates[i - 1]:\n continue\n if candidates[i] > target:\n break\n backtrack(i + 1, path + [candidates[i]], target - candidates[i])\n\n candidates.sort()\n res = []\n backtrack(0, [], target)\n return res\n\n``` | 2 | Given a collection of candidate numbers (`candidates`) and a target number (`target`), find all unique combinations in `candidates` where the candidate numbers sum to `target`.
Each number in `candidates` may only be used **once** in the combination.
**Note:** The solution set must not contain duplicate combinations.
**Example 1:**
**Input:** candidates = \[10,1,2,7,6,1,5\], target = 8
**Output:**
\[
\[1,1,6\],
\[1,2,5\],
\[1,7\],
\[2,6\]
\]
**Example 2:**
**Input:** candidates = \[2,5,2,1,2\], target = 5
**Output:**
\[
\[1,2,2\],
\[5\]
\]
**Constraints:**
* `1 <= candidates.length <= 100`
* `1 <= candidates[i] <= 50`
* `1 <= target <= 30` | null |
Python || BackTracking || Easy To Understand 🔥 | combination-sum-ii | 0 | 1 | \n# Code\n```\nclass Solution:\n def combinationSum2(self, candidates, target):\n candidates.sort() \n result = []\n def combine_sum_2(nums, start, path, result, target):\n if not target:\n result.append(path)\n return\n for i in range(start, len(nums)):\n if i > start and nums[i] == nums[i - 1]:\n continue\n if nums[i] > target:\n break\n combine_sum_2(nums, i + 1, path + [nums[i]], result, target - nums[i])\n combine_sum_2(candidates, 0, [], result, target)\n return result\n``` | 2 | Given a collection of candidate numbers (`candidates`) and a target number (`target`), find all unique combinations in `candidates` where the candidate numbers sum to `target`.
Each number in `candidates` may only be used **once** in the combination.
**Note:** The solution set must not contain duplicate combinations.
**Example 1:**
**Input:** candidates = \[10,1,2,7,6,1,5\], target = 8
**Output:**
\[
\[1,1,6\],
\[1,2,5\],
\[1,7\],
\[2,6\]
\]
**Example 2:**
**Input:** candidates = \[2,5,2,1,2\], target = 5
**Output:**
\[
\[1,2,2\],
\[5\]
\]
**Constraints:**
* `1 <= candidates.length <= 100`
* `1 <= candidates[i] <= 50`
* `1 <= target <= 30` | null |
Python || 99.53% Faster || Backtracking || Easy | combination-sum-ii | 0 | 1 | ```\nclass Solution:\n def combinationSum2(self, candidates: List[int], target: int) -> List[List[int]]:\n def solve(ind,target):\n if target==0:\n ans.append(op[:])\n return \n for i in range(ind,len(candidates)):\n if i>ind and candidates[i]==candidates[i-1]:\n continue\n if candidates[i]>target:\n break\n op.append(candidates[i])\n solve(i+1,target-candidates[i])\n op.pop()\n candidates.sort()\n ans=[]\n op=[]\n solve(0,target)\n return ans\n```\n**An upvote will be encouraging** | 3 | Given a collection of candidate numbers (`candidates`) and a target number (`target`), find all unique combinations in `candidates` where the candidate numbers sum to `target`.
Each number in `candidates` may only be used **once** in the combination.
**Note:** The solution set must not contain duplicate combinations.
**Example 1:**
**Input:** candidates = \[10,1,2,7,6,1,5\], target = 8
**Output:**
\[
\[1,1,6\],
\[1,2,5\],
\[1,7\],
\[2,6\]
\]
**Example 2:**
**Input:** candidates = \[2,5,2,1,2\], target = 5
**Output:**
\[
\[1,2,2\],
\[5\]
\]
**Constraints:**
* `1 <= candidates.length <= 100`
* `1 <= candidates[i] <= 50`
* `1 <= target <= 30` | null |
Easy Solution | first-missing-positive | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom typing import List\n\nclass Solution:\n def firstMissingPositive(self, nums: List[int]) -> int:\n n = len(nums)\n missing_value = n + 1\n\n for i in range(n):\n while 1 <= nums[i] <= n and nums[nums[i] - 1] != nums[i]:\n nums[nums[i] - 1], nums[i] = nums[i], nums[nums[i] - 1]\n\n for i in range(n):\n if nums[i] != i + 1:\n return i + 1\n\n return missing_value\n ##Upvote me IF it Helps\n\n``` | 3 | Given an unsorted integer array `nums`, return the smallest missing positive integer.
You must implement an algorithm that runs in `O(n)` time and uses constant extra space.
**Example 1:**
**Input:** nums = \[1,2,0\]
**Output:** 3
**Explanation:** The numbers in the range \[1,2\] are all in the array.
**Example 2:**
**Input:** nums = \[3,4,-1,1\]
**Output:** 2
**Explanation:** 1 is in the array but 2 is missing.
**Example 3:**
**Input:** nums = \[7,8,9,11,12\]
**Output:** 1
**Explanation:** The smallest positive integer 1 is missing.
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1` | Think about how you would solve the problem in non-constant space. Can you apply that logic to the existing space? We don't care about duplicates or non-positive integers Remember that O(2n) = O(n) |
BEATS 90% of the user ! Simplest answer . | first-missing-positive | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def firstMissingPositive(self, nums: List[int]) -> int:\n d={}\n for i in nums:\n d[i] = 1\n i=1\n while(True):\n if i not in d:\n return i\n i=i+1 \n``` | 0 | Given an unsorted integer array `nums`, return the smallest missing positive integer.
You must implement an algorithm that runs in `O(n)` time and uses constant extra space.
**Example 1:**
**Input:** nums = \[1,2,0\]
**Output:** 3
**Explanation:** The numbers in the range \[1,2\] are all in the array.
**Example 2:**
**Input:** nums = \[3,4,-1,1\]
**Output:** 2
**Explanation:** 1 is in the array but 2 is missing.
**Example 3:**
**Input:** nums = \[7,8,9,11,12\]
**Output:** 1
**Explanation:** The smallest positive integer 1 is missing.
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1` | Think about how you would solve the problem in non-constant space. Can you apply that logic to the existing space? We don't care about duplicates or non-positive integers Remember that O(2n) = O(n) |
✅ 100% Solved | Both - Very Simple way and Optimized way to solve Trapping rain water problem. | trapping-rain-water | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe code calculates trapped rainwater by iterating through each element in the input array. For each element, it determines the minimum height of barriers on both sides and calculates the trapped water if the minimum height exceeds the current height. The total trapped water is returned. **However, this simple code can be optimized for efficiency. please find optimized code at bottom setion**\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n* Travel the array from 1 to len(height)\n\n ``` \n for i in range ( 1 , len(height) ):\n ```\n* Find Left side maximum buiding height till \'i\' index\n ```\n left_max = max( height [ 0 : i ] )\n ```\n* Find Right side maximum building height from \'i\' index\n ```\n right_max = max ( height [ i : len(height) ] )\n ```\n* Find minimum height from left_max and right_max\n ```\n min_height = min( left_max , right_max )\n ```\n* If minimum height - height[i] is greater than 0 then add that values to water varieble by substracting element which is at \'i\' index.\n ```\n if(min_height - height[i]) > 0):\n water += ( min_height - height[i] )```\n* Finally return the total stored water\n ``` \n return water\n ```\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n For simple code: O(n^2)\n For Optimized code : O(n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n For simple code: O(n)\n For Optimized code : O(n)\n# Code\n```\nclass Solution:\n def trap(self, height: List[int]) -> int:\n water = 0 \n for i in range(1, len(height)):\n min_height = min( max(height[0:i]), max(height[i:len(height)]))\n if((min_height - height[i]) > 0):\n water += (min_height - height[i])\n return water\n```\n\n\n# Optimized Code with O(n) time complexity:\n```\nclass Solution:\n def trap(self, height: List[int]) -> int:\n if not height:\n return 0\n \n left_max, right_max = 0, 0\n left, right = 0, len(height) - 1\n water = 0\n \n while left < right:\n if height[left] < height[right]:\n if height[left] > left_max:\n left_max = height[left]\n else:\n water += left_max - height[left]\n left += 1\n else:\n if height[right] > right_max:\n right_max = height[right]\n else:\n water += right_max - height[right]\n right -= 1\n \n return water\n```\n\n=> Please comment, If you have any questions.\n\n\u2764 Please upvote if you found this usefull \u2764\n\n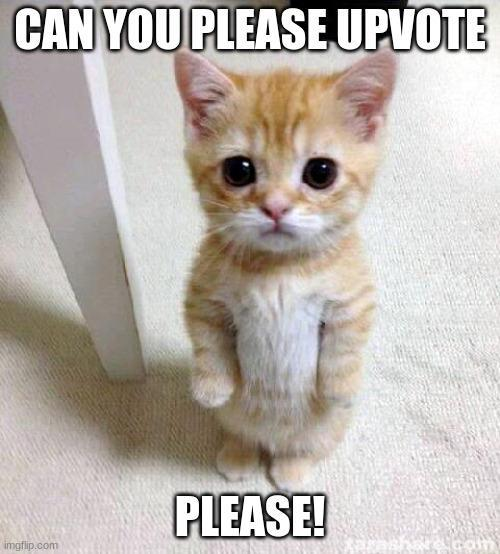\n\n\n | 30 | Given `n` non-negative integers representing an elevation map where the width of each bar is `1`, compute how much water it can trap after raining.
**Example 1:**
**Input:** height = \[0,1,0,2,1,0,1,3,2,1,2,1\]
**Output:** 6
**Explanation:** The above elevation map (black section) is represented by array \[0,1,0,2,1,0,1,3,2,1,2,1\]. In this case, 6 units of rain water (blue section) are being trapped.
**Example 2:**
**Input:** height = \[4,2,0,3,2,5\]
**Output:** 9
**Constraints:**
* `n == height.length`
* `1 <= n <= 2 * 104`
* `0 <= height[i] <= 105` | null |
Easy Solution | trapping-rain-water | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom typing import List\n\nclass Solution:\n def trap(self, height: List[int]) -> int:\n if len(height) <= 2:\n return 0\n\n ans = 0\n i, j = 1, len(height) - 1\n lmax, rmax = height[0], height[-1]\n\n while i <= j:\n # Update left and right maximum for the current positions\n if height[i] > lmax:\n lmax = height[i]\n if height[j] > rmax:\n rmax = height[j]\n\n # Fill water up to lmax level for index i and move i to the right\n if lmax <= rmax:\n ans += lmax - height[i]\n i += 1\n # Fill water up to rmax level for index j and move j to the left\n else:\n ans += rmax - height[j]\n j -= 1\n\n return ans\n ##Upvote me if it Helps\n\n``` | 5 | Given `n` non-negative integers representing an elevation map where the width of each bar is `1`, compute how much water it can trap after raining.
**Example 1:**
**Input:** height = \[0,1,0,2,1,0,1,3,2,1,2,1\]
**Output:** 6
**Explanation:** The above elevation map (black section) is represented by array \[0,1,0,2,1,0,1,3,2,1,2,1\]. In this case, 6 units of rain water (blue section) are being trapped.
**Example 2:**
**Input:** height = \[4,2,0,3,2,5\]
**Output:** 9
**Constraints:**
* `n == height.length`
* `1 <= n <= 2 * 104`
* `0 <= height[i] <= 105` | null |
Easy Python Solution) | trapping-rain-water | 0 | 1 | \n# Code\n```\nclass Solution:\n def trap(self, height: List[int]) -> int:\n left = 0\n right = len(height) - 1\n leftMax = 0\n rightMax = 0\n bucket = 0\n\n while left < right:\n if height[left] < height[right]:\n if leftMax < height[left]:\n leftMax = height[left]\n else:\n bucket += leftMax - height[left]\n left += 1\n else:\n if rightMax < height[right]:\n rightMax = height[right]\n else:\n bucket += rightMax - height[right]\n right -= 1\n \n return bucket\n``` | 0 | Given `n` non-negative integers representing an elevation map where the width of each bar is `1`, compute how much water it can trap after raining.
**Example 1:**
**Input:** height = \[0,1,0,2,1,0,1,3,2,1,2,1\]
**Output:** 6
**Explanation:** The above elevation map (black section) is represented by array \[0,1,0,2,1,0,1,3,2,1,2,1\]. In this case, 6 units of rain water (blue section) are being trapped.
**Example 2:**
**Input:** height = \[4,2,0,3,2,5\]
**Output:** 9
**Constraints:**
* `n == height.length`
* `1 <= n <= 2 * 104`
* `0 <= height[i] <= 105` | null |
Algorithm in Python (Beats 80%) | trapping-rain-water | 0 | 1 | ## Trapping Rain Water\n\nGiven an array of non-negative integers representing an elevation map where the width of each bar is 1, compute how much water it can trap after raining.\n\n### Approach:\n\nWe can solve this problem by maintaining two arrays, `l` and `r`, which represent the maximum height of bars to the left and right of each bar, respectively. Then, for each bar, we calculate how much water it can trap by taking the minimum of the maximum heights to its left and right and subtracting its own height.\n\n1. Initialize arrays `l` and `r`, both of size `n`, where `n` is the length of the input `height` array, with all elements initially set to 0.\n2. Initialize two variables, `lm` and `rm`, to 0. These variables will keep track of the maximum height encountered while traversing from left to right and right to left, respectively.\n3. Traverse the `height` array from left to right, and for each element `height[i]`, update `l[i]` to be equal to `lm` and update `lm` if `height[i]` is greater than `lm`.\n4. Traverse the `height` array from right to left, and for each element `height[i]`, update `r[i]` to be equal to `rm` and update `rm` if `height[i]` is greater than `rm`.\n5. Initialize a variable `ans` to 0. This variable will store the total trapped water.\n6. Traverse the `height` array again, and for each element `height[i]`, calculate the amount of trapped water as `min(l[i], r[i]) - height[i]`. If this value is greater than 0, add it to `ans`.\n7. Return `ans` as the final result.\n\n### Complexity Analysis:\n\n- Time Complexity: O(n)\n - We traverse the `height` array three times, each time taking O(n) operations. Hence, the overall time complexity is O(n).\n\n- Space Complexity: O(n)\n - We use two additional arrays, `l` and `r`, of size `n`, and a few integer variables. Therefore, the space complexity is O(n).\n\n\n# Code\n```\nclass Solution:\n def trap(self, height: List[int]) -> int:\n n = len(height)\n l = [0] * n \n r = [0] * n\n ans = 0\n lm, rm = 0, 0\n\n for i in range(n):\n l[i] = lm\n if lm < height[i]:\n lm = height[i]\n for i in range(n - 1, -1, -1):\n r[i] = rm\n if rm < height[i]:\n rm = height[i]\n for i in range(n):\n trapped = min(l[i], r[i]) - height[i]\n if trapped > 0:\n ans += trapped\n\n return ans\n\n```\n\n# Please upvote the solution if you understood it.\n\n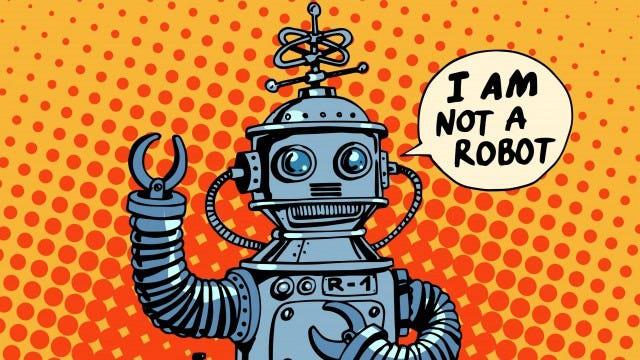\n\n | 7 | Given `n` non-negative integers representing an elevation map where the width of each bar is `1`, compute how much water it can trap after raining.
**Example 1:**
**Input:** height = \[0,1,0,2,1,0,1,3,2,1,2,1\]
**Output:** 6
**Explanation:** The above elevation map (black section) is represented by array \[0,1,0,2,1,0,1,3,2,1,2,1\]. In this case, 6 units of rain water (blue section) are being trapped.
**Example 2:**
**Input:** height = \[4,2,0,3,2,5\]
**Output:** 9
**Constraints:**
* `n == height.length`
* `1 <= n <= 2 * 104`
* `0 <= height[i] <= 105` | null |
Easy to understand 99% fast method (Python) | trapping-rain-water | 0 | 1 | # Intuition\n\nTo solve this problem, imagine that the map is a pyramid.\nWe have steps up to the column with the highest height.\nAfter the peak with the maximum height, we have steps going down.\nRight.\nNow we need to calculating total sum of bars with water.\n\n# Approach\n\nFirst step - for i in range(1,listLen):\nIn this loop we try to calculate sum of water bars that are located to the left of the column rith max high\n\nSecond step - for i in range(listLen-1, indexMax, -1):\nIn this loop we try to calculate sum of water bars that are located to the right of the column rith max high\n\n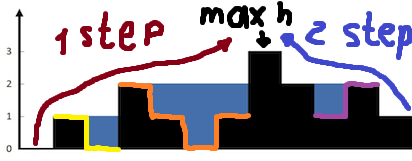\n\nIn the loop we try to find nowMax that mean the highest column of i - step\nThen if we find on i+n - step the column with high $$<$$ that the nowMax\nWe can say that total sum of water bars $$=$$ nowMax - high on i+n step \n\n# Complexity\n- Time complexity:\n$$O(2N) => O(N)$$\n\n# Code\n```\nclass Solution:\n def trap(self, height: List[int]) -> int:\n indexMax = 0\n listLen = len(height)\n total = 0\n for i in range(1,listLen):\n if height[i] > height[indexMax]:\n indexMax = i\n nowMax = height[0]\n for i in range(1, indexMax):\n if height[i] > nowMax:\n nowMax = height[i]\n else:\n total += (nowMax-height[i])\n nowMax = height[listLen-1]\n for i in range(listLen-1, indexMax, -1):\n if height[i] > nowMax:\n nowMax = height[i]\n else:\n total += (nowMax-height[i])\n return total\n``` | 5 | Given `n` non-negative integers representing an elevation map where the width of each bar is `1`, compute how much water it can trap after raining.
**Example 1:**
**Input:** height = \[0,1,0,2,1,0,1,3,2,1,2,1\]
**Output:** 6
**Explanation:** The above elevation map (black section) is represented by array \[0,1,0,2,1,0,1,3,2,1,2,1\]. In this case, 6 units of rain water (blue section) are being trapped.
**Example 2:**
**Input:** height = \[4,2,0,3,2,5\]
**Output:** 9
**Constraints:**
* `n == height.length`
* `1 <= n <= 2 * 104`
* `0 <= height[i] <= 105` | null |
Easy solution using 2 lists in python | trapping-rain-water | 0 | 1 | ```\nclass Solution:\n def trap(self, height: List[int]) -> int:\n data=[0 for i in range(len(height))]\n c=0\n front=[height[0]]\n back=[height[-1]]\n r=len(height)\n for i in range(1,len(height)):\n front.append(max(front[i-1],height[i]))\n back.append(max(back[i-1],height[-1*(i+1)]))\n for i in range(len(height)):\n m=min(front[i],back[-1*(i+1)])-height[i]\n c+=m\n return c\n``` | 2 | Given `n` non-negative integers representing an elevation map where the width of each bar is `1`, compute how much water it can trap after raining.
**Example 1:**
**Input:** height = \[0,1,0,2,1,0,1,3,2,1,2,1\]
**Output:** 6
**Explanation:** The above elevation map (black section) is represented by array \[0,1,0,2,1,0,1,3,2,1,2,1\]. In this case, 6 units of rain water (blue section) are being trapped.
**Example 2:**
**Input:** height = \[4,2,0,3,2,5\]
**Output:** 9
**Constraints:**
* `n == height.length`
* `1 <= n <= 2 * 104`
* `0 <= height[i] <= 105` | null |
Python3- Easy to understand | trapping-rain-water | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def trap(self, arr: List[int]) -> int:\n left,right=[],[]\n mx,mx1,n=0,0,len(arr)\n for i in arr:\n mx=max(mx,i)\n left.append(mx)\n for i in range(n-1,-1,-1):\n mx1=max(mx1,arr[i])\n right.append(mx1)\n right=right[::-1]\n list1=[]\n for i,j in zip(left,right):\n list1.append(min(i,j))\n count=0\n for i in range(n):\n if list1[i]-arr[i]>0:\n count+=list1[i]-arr[i]\n return count\n``` | 1 | Given `n` non-negative integers representing an elevation map where the width of each bar is `1`, compute how much water it can trap after raining.
**Example 1:**
**Input:** height = \[0,1,0,2,1,0,1,3,2,1,2,1\]
**Output:** 6
**Explanation:** The above elevation map (black section) is represented by array \[0,1,0,2,1,0,1,3,2,1,2,1\]. In this case, 6 units of rain water (blue section) are being trapped.
**Example 2:**
**Input:** height = \[4,2,0,3,2,5\]
**Output:** 9
**Constraints:**
* `n == height.length`
* `1 <= n <= 2 * 104`
* `0 <= height[i] <= 105` | null |
Short solution that beats 80% (python) | trapping-rain-water | 0 | 1 | # Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nclass Solution:\n def trap(self, height: List[int]) -> int:\n curr_max = 0\n max_left = [curr_max:= max(curr_max, h) for h in height]\n curr_max = 0\n max_right = [curr_max:= max(curr_max, h) for h in reversed(height)]\n max_right.reverse()\n return sum([min(max_left[i], max_right[i]) - height[i] for i in range(len(height))])\n \n``` | 3 | Given `n` non-negative integers representing an elevation map where the width of each bar is `1`, compute how much water it can trap after raining.
**Example 1:**
**Input:** height = \[0,1,0,2,1,0,1,3,2,1,2,1\]
**Output:** 6
**Explanation:** The above elevation map (black section) is represented by array \[0,1,0,2,1,0,1,3,2,1,2,1\]. In this case, 6 units of rain water (blue section) are being trapped.
**Example 2:**
**Input:** height = \[4,2,0,3,2,5\]
**Output:** 9
**Constraints:**
* `n == height.length`
* `1 <= n <= 2 * 104`
* `0 <= height[i] <= 105` | null |
this problem solve | multiply-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def multiply(self, num1: str, num2: str) -> str:\n\n sys.set_int_max_str_digits(10000)\n val1 = int(num1)\n val2 = int(num2)\n result = str(val1 *val2)\n return result\n``` | 0 | Given two non-negative integers `num1` and `num2` represented as strings, return the product of `num1` and `num2`, also represented as a string.
**Note:** You must not use any built-in BigInteger library or convert the inputs to integer directly.
**Example 1:**
**Input:** num1 = "2", num2 = "3"
**Output:** "6"
**Example 2:**
**Input:** num1 = "123", num2 = "456"
**Output:** "56088"
**Constraints:**
* `1 <= num1.length, num2.length <= 200`
* `num1` and `num2` consist of digits only.
* Both `num1` and `num2` do not contain any leading zero, except the number `0` itself. | null |
Readable Python solution | multiply-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def multiply(self, num1: str, num2: str) -> str:\n a = 0\n b = 0\n count = -1\n for indd, d in enumerate(num1):\n while str(count) != d:\n count += 1\n a += count*10**(len(num1)-1-indd)\n count = -1\n for indz, z in enumerate(num2):\n while str(count) != z:\n count += 1\n b += count*10**(len(num2)-1-indz)\n count = -1\n return str(a*b)\n``` | 1 | Given two non-negative integers `num1` and `num2` represented as strings, return the product of `num1` and `num2`, also represented as a string.
**Note:** You must not use any built-in BigInteger library or convert the inputs to integer directly.
**Example 1:**
**Input:** num1 = "2", num2 = "3"
**Output:** "6"
**Example 2:**
**Input:** num1 = "123", num2 = "456"
**Output:** "56088"
**Constraints:**
* `1 <= num1.length, num2.length <= 200`
* `num1` and `num2` consist of digits only.
* Both `num1` and `num2` do not contain any leading zero, except the number `0` itself. | null |
Python3 || Beats 10% :( | multiply-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def multiply(self, num1: str, num2: str) -> str:\n l1=len(num1)\n l2=len(num2)\n\n if (l2>l1): # taking smaller string as nums2\n num1,num2=num2,num1\n\n l1=len(num1)\n l2=len(num2)\n\n res=[0]*(l1+l2)\n res_pointer=len(res)-1\n res_pointer_count=0\n\n carry=0\n\n j=l2-1\n\n while j>-1:\n res_pointer=len(res)-1-res_pointer_count\n for i in range((l1)-1,-1,-1):\n temp1 = int(num1[i])\n temp2 = int(num2[j])\n\n inplace = (temp1*temp2)%10\n carry= (temp1*temp2)//10\n\n res[res_pointer]+=inplace\n res[res_pointer-1]+=carry\n\n if res[res_pointer]>9:\n inplace = res[res_pointer]%10\n carry= res[res_pointer]//10\n res[res_pointer]=inplace\n res[res_pointer-1]+=carry\n\n res_pointer-=1\n\n res_pointer_count+=1\n j-=1\n\n ans=""\n for i in res:\n ans=ans+str(i)\n return str(int(ans))\n``` | 5 | Given two non-negative integers `num1` and `num2` represented as strings, return the product of `num1` and `num2`, also represented as a string.
**Note:** You must not use any built-in BigInteger library or convert the inputs to integer directly.
**Example 1:**
**Input:** num1 = "2", num2 = "3"
**Output:** "6"
**Example 2:**
**Input:** num1 = "123", num2 = "456"
**Output:** "56088"
**Constraints:**
* `1 <= num1.length, num2.length <= 200`
* `num1` and `num2` consist of digits only.
* Both `num1` and `num2` do not contain any leading zero, except the number `0` itself. | null |
Python one-liner top 5% | multiply-strings | 0 | 1 | # Approach\nSince we\'re using dynamically-typed language - we can convert a string into an integer, perform our calculations and convert the solution back to string in just one line, using built-in library :D\n\n\n# Code\n```\nclass Solution:\n def multiply(self, num1: str, num2: str) -> str:\n return str(int(num1)*int(num2))\n``` | 0 | Given two non-negative integers `num1` and `num2` represented as strings, return the product of `num1` and `num2`, also represented as a string.
**Note:** You must not use any built-in BigInteger library or convert the inputs to integer directly.
**Example 1:**
**Input:** num1 = "2", num2 = "3"
**Output:** "6"
**Example 2:**
**Input:** num1 = "123", num2 = "456"
**Output:** "56088"
**Constraints:**
* `1 <= num1.length, num2.length <= 200`
* `num1` and `num2` consist of digits only.
* Both `num1` and `num2` do not contain any leading zero, except the number `0` itself. | null |
Easy Python Solution without Using Dictionary | multiply-strings | 0 | 1 | ```\nclass Solution:\n def multiply(self, num1: str, num2: str) -> str:\n if num1 == \'0\' or num2 == \'0\':\n return \'0\'\n \n def decode(num):\n ans = 0\n for i in num:\n ans = ans*10 +(ord(i) - ord(\'0\'))\n return ans\n\n def encode(s):\n news = \'\'\n while s:\n a = s % 10\n s = s // 10\n news = chr(ord(\'0\') + a) + news\n return news\n \n return encode(decode(num1)*decode(num2))\n``` | 47 | Given two non-negative integers `num1` and `num2` represented as strings, return the product of `num1` and `num2`, also represented as a string.
**Note:** You must not use any built-in BigInteger library or convert the inputs to integer directly.
**Example 1:**
**Input:** num1 = "2", num2 = "3"
**Output:** "6"
**Example 2:**
**Input:** num1 = "123", num2 = "456"
**Output:** "56088"
**Constraints:**
* `1 <= num1.length, num2.length <= 200`
* `num1` and `num2` consist of digits only.
* Both `num1` and `num2` do not contain any leading zero, except the number `0` itself. | null |
Python3 || Beats 96.57% || One liner beginner solution. | multiply-strings | 0 | 1 | # Code\n```\nclass Solution:\n def multiply(self, num1: str, num2: str) -> str:\n return str(int(num1)*int(num2))\n```\n# Please do upvote if you like the solution. | 4 | Given two non-negative integers `num1` and `num2` represented as strings, return the product of `num1` and `num2`, also represented as a string.
**Note:** You must not use any built-in BigInteger library or convert the inputs to integer directly.
**Example 1:**
**Input:** num1 = "2", num2 = "3"
**Output:** "6"
**Example 2:**
**Input:** num1 = "123", num2 = "456"
**Output:** "56088"
**Constraints:**
* `1 <= num1.length, num2.length <= 200`
* `num1` and `num2` consist of digits only.
* Both `num1` and `num2` do not contain any leading zero, except the number `0` itself. | null |
Python3 | multiply-strings | 0 | 1 | Ru\n## \u0418\u0434\u0435\u044F\n\u041D\u0443\u0436\u043D\u043E \u0441\u043E\u0437\u0434\u0430\u0442\u044C \u043C\u0430\u0441\u0441\u0438\u0432 \u0434\u043B\u0438\u043D\u043E\u0439 n+m, \u0433\u0434\u0435 n \u0438 m - \u0434\u043B\u0438\u043D\u044B \u0441\u0442\u0440\u043E\u043A num1 \u0438 num2 \u0441\u043E\u043E\u0442\u0432\u0435\u0442\u0441\u0442\u0432\u0435\u043D\u043D\u043E. \u0417\u0430\u0442\u0435\u043C \u043C\u044B \u043D\u0430\u0447\u0438\u043D\u0430\u0435\u043C \u0443\u043C\u043D\u043E\u0436\u0435\u043D\u0438\u0435, \u043D\u0430\u0447\u0438\u043D\u0430\u044F \u0441 \u043A\u043E\u043D\u0446\u0430 \u043A\u0430\u0436\u0434\u043E\u0433\u043E \u0447\u0438\u0441\u043B\u0430, \u0438 \u043F\u0435\u0440\u0435\u043C\u0435\u0449\u0430\u0435\u043C\u0441\u044F \u0432\u043B\u0435\u0432\u043E. \u041C\u044B \u0441\u043E\u0445\u0440\u0430\u043D\u044F\u0435\u043C \u0440\u0435\u0437\u0443\u043B\u044C\u0442\u0430\u0442 \u043A\u0430\u0436\u0434\u043E\u0433\u043E \u0443\u043C\u043D\u043E\u0436\u0435\u043D\u0438\u044F \u0432 \u044F\u0447\u0435\u0439\u043A\u0435 \u043C\u0430\u0441\u0441\u0438\u0432\u0430, \u043D\u0430\u0447\u0438\u043D\u0430\u044E\u0449\u0435\u0439\u0441\u044F \u0441 \u0441\u043E\u043E\u0442\u0432\u0435\u0442\u0441\u0442\u0432\u0443\u044E\u0449\u0435\u0433\u043E \u0438\u043D\u0434\u0435\u043A\u0441\u0430.\n\n\u041F\u043E\u0441\u043B\u0435 \u044D\u0442\u043E\u0433\u043E \u043C\u044B \u043C\u043E\u0436\u0435\u043C \u043F\u043E\u043B\u0443\u0447\u0438\u0442\u044C \u043E\u043A\u043E\u043D\u0447\u0430\u0442\u0435\u043B\u044C\u043D\u044B\u0439 \u0440\u0435\u0437\u0443\u043B\u044C\u0442\u0430\u0442, \u043F\u0440\u043E\u0445\u043E\u0434\u044F\u0441\u044C \u043F\u043E \u043C\u0430\u0441\u0441\u0438\u0432\u0443, \u043D\u0430\u0447\u0438\u043D\u0430\u044F \u0441 \u043F\u0435\u0440\u0432\u043E\u0433\u043E \u0438\u043D\u0434\u0435\u043A\u0441\u0430, \u0438 \u043E\u0431\u044A\u0435\u0434\u0438\u043D\u044F\u0442\u044C \u0432\u0441\u0435 \u0447\u0438\u0441\u043B\u0430 \u0432 \u0441\u0442\u0440\u043E\u043A\u0443. \u0415\u0441\u043B\u0438 \u0440\u0435\u0437\u0443\u043B\u044C\u0442\u0430\u0442 \u0440\u0430\u0432\u0435\u043D \u043D\u0443\u043B\u044E, \u043C\u044B \u0434\u043E\u043B\u0436\u043D\u044B \u0432\u0435\u0440\u043D\u0443\u0442\u044C \u0441\u0442\u0440\u043E\u043A\u0443 "0".\n\n# Code\n```\nclass Solution:\n def multiply(self, num1: str, num2: str) -> str:\n m, n = len(num1), len(num2)\n products = [0] * (m + n)\n for i in range(m-1, -1, -1):\n for j in range(n-1, -1, -1):\n digit1 = ord(num1[i]) - ord(\'0\')\n digit2 = ord(num2[j]) - ord(\'0\')\n product = digit1 * digit2\n pos1, pos2 = i+j, i+j+1\n carry, value = divmod(product + products[pos2], 10)\n products[pos1] += carry\n products[pos2] = value\n \n result = ""\n for digit in products:\n if not (len(result) == 0 and digit == 0):\n result += str(digit)\n return result if len(result) > 0 else "0"\n``` | 1 | Given two non-negative integers `num1` and `num2` represented as strings, return the product of `num1` and `num2`, also represented as a string.
**Note:** You must not use any built-in BigInteger library or convert the inputs to integer directly.
**Example 1:**
**Input:** num1 = "2", num2 = "3"
**Output:** "6"
**Example 2:**
**Input:** num1 = "123", num2 = "456"
**Output:** "56088"
**Constraints:**
* `1 <= num1.length, num2.length <= 200`
* `num1` and `num2` consist of digits only.
* Both `num1` and `num2` do not contain any leading zero, except the number `0` itself. | null |
Simple Python Solution beats 90% | multiply-strings | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def multiply(self, num1: str, num2: str) -> str:\n dic={\n \'1\':1,\n \'2\':2,\n \'3\':3,\n \'4\':4,\n \'5\':5,\n \'6\':6,\n \'7\':7,\n \'8\':8,\n \'9\':9,\n \'0\':0\n }\n h=list(num1)\n l=list(num2)\n s=len(num1)-1\n s1=len(l)-1\n k=0\n k1=0\n p=0\n p1=0\n while h:\n r=h.pop(s)\n p1+=(10**k)*dic[r]\n k+=1\n s-=1\n while l:\n r=l.pop(s1)\n p+=(10**k1)*dic[r]\n k1+=1\n s1-=1\n return str((p1*p))\n \n``` | 2 | Given two non-negative integers `num1` and `num2` represented as strings, return the product of `num1` and `num2`, also represented as a string.
**Note:** You must not use any built-in BigInteger library or convert the inputs to integer directly.
**Example 1:**
**Input:** num1 = "2", num2 = "3"
**Output:** "6"
**Example 2:**
**Input:** num1 = "123", num2 = "456"
**Output:** "56088"
**Constraints:**
* `1 <= num1.length, num2.length <= 200`
* `num1` and `num2` consist of digits only.
* Both `num1` and `num2` do not contain any leading zero, except the number `0` itself. | null |
Subsets and Splits