branch_name
stringclasses 15
values | target
stringlengths 26
10.3M
| directory_id
stringlengths 40
40
| languages
sequencelengths 1
9
| num_files
int64 1
1.47k
| repo_language
stringclasses 34
values | repo_name
stringlengths 6
91
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
| input
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|
refs/heads/gh-pages | <file_sep># ACA-todo-list.js
<file_sep>'use strict';
$(document).ready(function() {
// You code here
$.ajax('https://reqres-api.herokuapp.com/api/users/', {
success: function successHandler(response) {
$('tbody').empty();
response.forEach(function(user) {
var $str = "<tr><td>" + user.id + "</td><td>" + user.first_name + "</td><td>" + user.last_name + "</td><td><a href='#' data-id='" + user.id + "'>View</a></td></tr>";
$("tbody").append($str);
});
$('tbody tr td a').click(function(event) {
event.preventDefault();
var $id = $(this).data('id');
var $url = "https://reqres-api.herokuapp.com/api/users/" + $id;
$.ajax($url,{
success: function(person){
var $string = $("<h2>" + person.first_name + ' '+ person.last_name + "</h2><h4>" + person.occupation + "</h4><p>" + person.phone +"</p><p>" + person.address + "</p><img src='"+ person.avatar+ "'>");
$('#details').html($string);
}
})
});
}
});
});
<file_sep># ACA-towers-app.js
<file_sep>'use strict';
// lets the application know that the page is loaded and ready to execute the code
$(document).on('ready', function() {
// Put app logic in here
// how you get all of the divs with "data" in the element tag
//.click() - when you click these things (anything)
var $datacell = $('[data-cell]');
var playerTurn = "X";
var $button = $('#clear');
//html board layout:
//0 1 2
//3 4 5
//6 7 8
//how to loop thorugh winning combos this would need to go in the check for win function
// var winningcombo = [[0,1,2], [2,3,4]]
//
// for (var i =0; i < winningcombo.length, i++) {
// if(
//
// $('[data-cell="'+ winningcombo[i][0]'"]') === playerTurn &&
// $('[data-cell="'+ winningcombo[i][1]'"]') === playerTurn &&
// $('[data-cell="'+ winningcombo[i][2]'"]') === playerTurn){
// return true;
// }
// }
var datacells0 = $('[data-cell="0"]').text();
var datacells1 = $('[data-cell="1"]').text();
var datacells2 = $('[data-cell="2"]').text();
var datacells3 = $('[data-cell="3"]').text();
var datacells4 = $('[data-cell="4"]').text();
var datacells5 = $('[data-cell="5"]').text();
var datacells6 = $('[data-cell="6"]').text();
var datacells7 = $('[data-cell="7"]').text();
var datacells8 = $('[data-cell="8"]').text();
//Using jQuery, set a click listener on all of the [data-cell]s that SETs playerTurn as .text() on $(this) by $(this).text(playerTurn)
$datacell.click(function() {
$(this).text(playerTurn)
checkForWin();
playerTurn = (playerTurn === "X") ? "O" : "X";
});
//Add a button with an id="clear" that will not only clear the board, but reset the player to player 'X'
$button.click(function() {
$datacell.empty();
$("#announce-winner").empty();
playerTurn = "X";
});
//
function horizontalWin() {
if (datacells0 === playerTurn && datacells1 === playerTurn && datacells2 === playerTurn ||
datacells3 === playerTurn && datacells4 === playerTurn && datacells5 === playerTurn ||
datacells6 === playerTurn && datacells7 === playerTurn && datacells8 === playerTurn) {
return true;
}
}
function verticalWin() {
if (datacells0 === playerTurn && datacells3 === playerTurn && datacells6 === playerTurn ||
datacells1 === playerTurn && datacells4 === playerTurn && datacells7 === playerTurn ||
datacells2 === playerTurn && datacells5 === playerTurn && datacells8 === playerTurn) {
return true;
}
}
function diagonalWin() {
console.log('test2: ' + datacells8);
if (datacells0 === playerTurn && datacells4 === playerTurn && datacells8 === playerTurn ||
datacells2 === playerTurn && datacells4 === playerTurn && datacells6 === playerTurn) {
return true;
}
}
function checkForWin() {
datacells0 = $('[data-cell="0"]').text();
datacells1 = $('[data-cell="1"]').text();
datacells2 = $('[data-cell="2"]').text();
datacells3 = $('[data-cell="3"]').text();
datacells4 = $('[data-cell="4"]').text();
datacells5 = $('[data-cell="5"]').text();
datacells6 = $('[data-cell="6"]').text();
datacells7 = $('[data-cell="7"]').text();
datacells8 = $('[data-cell="8"]').text();
if (horizontalWin() || verticalWin() || diagonalWin()) {
//need to select '#announce-winner' and SET its .text() to say 'Player ' + playerTurn + 'Wins!'
$('#announce-winner').text('Player ' + playerTurn + ' Wins!');
return true;
// process.exit();
}
}
});
<file_sep>'use strict';
$(document).ready(function() {
$.ajax("http://127.0.0.1:8080/apps/11gist-blog/api/gists.json", {
success: function(gists) {
console.log(gists);
gists.forEach(function(post) {
if (post.description.startsWith('#post')) {
var strPostDiscription = post.description.slice(5);
var $post = $(`<a href="#" data-url="${post.url}"
data-comments="${post.comments_url}" >${strPostDiscription}</a>`);
$("ul#posts").append($post);
}
$('ul#posts a').click(function(event) {
var $that = $(this).data('url').id;
console.log($that);
event.preventDefault();
$('ul#posts a').removeClass('active');
$(this).addClass('active');
$.ajax($(this).data('url'), {
success: function(post) {
var postContent = post.files['post.md'].content;
$('div#post').html(marked(postContent));
$('div#post p').attr('style', 'text-align: left');
}
});
$.ajax($(this).data('comments'), {
success: function(comments) {
$('ul#comments').empty();
comments.forEach(function(comment) {
var $comment = $('<blockquote><li><span class="comment-user">' + comment.user.login + '</span> says "<span class="comment-body">' + comment.body + '"</span></li></blockquote>');
$('#comments').append($comment);
})
}
})
});
});
});
});
}
// // Your code here
// So let's run through the API real quick. First, in your terminal, navigate to to your app directory:
// After starting up a server in the app directory, navigate in your browser to http://127.0.0.1:8080/apps/11gist-blog/api/gists.json, here you will see the top level of the api, with all of the gists. In each gist, you will see a "url" key. Navigate to that URL. In that address you will see another JSON object. Look for a "files" key with an object containing a file. In that file you'll see the "content" of the file. Next look for a "comments_url". At that address, you'll see a collection of comments, with keys such as "user" and "body".
// Spec 1.1
// Using jQuery to make an AJAX call, insert a list of links into #posts using JavaScript forEach with the "description" of each gist as the text.
// Spec 1.2
// Within each loop, write an if condition to insert only gists that start with a '#post' in the "description". After fitering, remove the '#post ' from the title.Spec 1.3
// Set the href attribute on each link to "#", and a "data-url" attribute equal to the "url" value.
// Spec 2
// After the links are are inserted, add a click listener, and prevent the default event from occuring. Then make an ajax call with the "data-url" value, grabbing it with $.data('url').
// Spec 3
// Insert the "content" of the file named post.md into #post.
// Spec 3.1
// Since our "content" is written in Markdown, we can use the Marked.js library to convert the content to html. Simply call marked() on your content. e.g. marked(content).
// Spec 4
// After inserting your content, make another ajax call using the "comments_url", and insert the ["user"]["login"] and "body" in a list in #comments.
// Spec 5
// Make it look nice! This is going to be YOUR blog! Take a look at some CSS frameworks, such as:
// Materialize - Framework based on Google's Material Design Guidelines
// Material Design Lite - Google's Official CSS Material Framework
// Bootstrap - Probably the most popular CSS framework on the web. Made by Twitter
// Foundation - Industry standard of CSS Frameworks
// Skeleton - Beautifully simple CSS Framework
// Spec 7
// After development and styling on your blog dies down, you can plug it into your Github account. If done correctly, you should only have to change one url: http://127.0.0.1:8080/apps/11gist-blog/api/gists.json to https://api.github.com/users/<your github username>/gists. Now whenever you create a gist with "#post" in the title and a file named post.md, it will automatically be pulled into your blog!
<file_sep>'use strict';
var assert = require('assert');
// ****
// Functions
// ****
// Problem 0:
// alwaysFalse() is a function that always
// returns the boolean value false
function alwaysFalse() {
return false;
}
// You can also define functions this way,
// by assigning function definitions to variable
var alwaysTrue = function() {
return true;
};
// Problem 1:
// equals(argument1, argument2) is an empty function
// return a boolean expression that is true when
// argument1 is equal to argument2
var argument1 = 5;
var argument2 = 4;
function equals(argument1, argument2) {
if (argument1 === argument2) {
return true;
} else {
return false;
}
}
// Problem 2:
// lessThanOrEqualTo(parameter1, parameter2) is an empty function
// return a boolean expression that is true when
// parameter1 is less than or equal to parameter2
var parameter1 = 1;
var parameter2 = 2;
function lessThanOrEqualTo(parameter1, parameter2) {
if (parameter1 <= parameter2) {
return true;
} else {
return false;
}
}
// Problem 3:
// write a function named add(number1, number2)
// add will add two numbers and return the result
var number1 = 1;
var number2 = 2;
function add(number1, number2) {
return number1 + number2;
}
var number3 = add(2, 4);
console.log(number3);
// Problem 4:
// write a function named addThree(number1, number2, number3)
// this function will add three numbers
// you must call your function add() in addThree()
function add(number1, number2) {
return number1 + number2;
}
function addThree(number1, number2, number3) {
return number1 + number2 + number3;
}
var addFive = add(1, 2) + addThree(3, 4, 5);
addFive;
// ****
// Concept Checkpoint
//
// Write your answer in comments
//
// What is a function? How do you define a function in Javascript?
//
// Your Answer Goes Here:
//In Javascript a function is a set of statements that performs a task or calculates a value.
// Functions are first-class objects. Like other objects, functions can have properties and methods.
// Functions can be called(invoked). This distinguishes them from other objects.
// //
// To define a function in Javascript we need the following:
// 1. The keyword function
// 2. The name of the function
// 3. A list of arguments for the function, seperated by commas and enclosed in parantheses.
// 4. The statement or statements (code to be executed) enclosed with curly brackets.
// //
//
// What is a return value?
//
// Your Answer Goes Here:
//The return statement stops the execution of a function and returns a value from that function. While updating global variables is one way to pass information back to the code that called the function , this is not an ideal way of doing so.
//
// How do you define a named function?
//
// Your Answer Goes Here:
//Named functions can be either declared in a statement or used in an expression. Named function expressions create readable stack traces. The name of the function is bound inside its body, and that can be useful. And we can use the name to have a function invoke itself, or to access its properties like any other object.
//
// What is a parameter? What is an argument? Is there a difference between the two?
// Your Answer Goes Here:
//// parameter-The Arguments Object. JavaScript functions have a built-in object called the arguments object. The argument object contains an array of the arguments used when the function was called (invoked).
// argument- The Arguments Object. JavaScript functions have a built-in object called the arguments object. The argument object contains an array of the arguments used when the function was called (invoked).
// A parameter is a variable in a method definition. When a method is called, the arguments are the data you pass into the method's parameters. Parameter is variable in the declaration of function. Argument is the actual value of this variable that gets passed to function.
// ****
// ****
// Modulo % Operator
// ****
// Problem 5:
// isEven(number) is a function that
// returns true if number is even (divisible by 2),
// else returns false
// complete isEven() by returning a boolean expression
var number = 8
function isEven(number) {
if (number % 2 === 0) {
return true;
} else {
return false;
}
}
// Problem 6:
// isDivisibleByThree(number) is a function that
// returns true if number is divisible by 3,
// else returns false
// complete isDivisibleByThree() by returning a boolean expression
var number = 9;
function isDivisibleByThree(number) {
if (number % 3 === 0) {
return true;
} else {
return false;
}
}
// ****
// Conditionals
// ****
// Problem 7:
// whichSpecies(character) is a function that
// should return "dog" when character is 'scooby'
// should return "cat" when character is 'garfield'
// should return "fish" when character is 'nemo'
// should return false if character is anything else
var character = 'scooby';
function whichSpecies(character) {
if (character === 'scooby') {
return ('dog');
} else if (character === 'garfield') {
return ('cat');
} else if (character === 'nemo') {
return ('fish');
} else {
return false;
}
}
// Problem 8:
// write a function named testNumber(number) with the following requirements.
// The function should:
// return the string "divisible by 4" when number % 4 === 0
// return the string "divisible by 2" when number % 2 === 0
// return the string "divisible by 3" when number % 3 === 0
// return the string "divisible by 5" when number % 5 === 0
var number = 8;
function testNumber(number){
if (number % 4 === 0){
return ('divisible by 4');
}
else if (number % 2 === 0){
return ('divisible by 2');
}
else if (number % 3 === 0){
return ('divisible by 3');
}
else if (number % 5 === 0){
return ('divisible by 5');
}
}
// ****
// Concept Checkpoint
//
// Write your answer in comments
//
// In your own words, explain what conditionals do.
//
// Your Answer Goes Here:
//
//
// ****
// ****
// Tests
// ****
describe('Lesson 2 Homework', function() {
describe('Functions', function() {
describe('Problem 0: alwaysFalse()', function() {
it('should return false', function() {
assert.equal(alwaysFalse(), false);
});
});
describe('Problem 0: alwaysTrue()', function() {
it('should return true', function() {
assert.equal(alwaysTrue(), true);
});
});
describe('Problem 1: equals()', function() {
it('should return true when argument 1 equals argument 2, else return false', function() {
assert.equal(equals(3, 3), true);
assert.equal(equals(3, null), false);
assert.equal(equals('bob', ''), false);
assert.equal(equals('bob', 'bob'), true);
});
});
describe('Problem 2: lessThanOrEqualTo()', function() {
it('should return true when parameter 1 is less than or equal to parameter 2, else return false', function() {
assert.equal(lessThanOrEqualTo(3, 3), true);
assert.equal(lessThanOrEqualTo(3, 4), true);
assert.equal(lessThanOrEqualTo(4, 1), false);
});
});
describe('Problem 3: add(number1, number2)', function() {
it('should be defined and of type function', function() {
assert(!(typeof add === 'undefined'));
assert(typeof add === 'function');
});
it('should add two numbers and return the result', function() {
assert.equal(add(3, 3), 6);
assert.equal(add(3, 7), 10);
});
});
describe('Problem 4: addThree(number1, number2, number3)', function() {
it('should be defined and of type function', function() {
assert(!(typeof addThree === 'undefined'));
assert(typeof addThree === 'function');
});
it('should add three numbers and return the result', function() {
assert.equal(addThree(3, 3, 3), 9);
});
});
});
describe('Modulo % operator', function() {
describe('Problem 5: isEven(number)', function() {
it('should return true if number is even, else false', function() {
assert.equal(isEven(4), true);
assert.equal(isEven(3), false);
});
});
describe('Problem 6: isDivisibleByThree(number)', function() {
it('should return true if number is divisible by 3, else false', function() {
assert.equal(isDivisibleByThree(3), true);
assert.equal(isDivisibleByThree(4), false);
});
});
});
describe('Conditionals', function() {
describe('Problem 7: whichSpecies(character)', function() {
it('should return "dog" when character is scooby', function() {
assert.equal(whichSpecies('scooby'), 'dog');
});
it('should return "cat" when character is garfield', function() {
assert.equal(whichSpecies('garfield'), 'cat');
});
it('should return "fish" when character is nemo', function() {
assert.equal(whichSpecies('nemo'), 'fish');
});
it('should return false if character is anything else', function() {
assert.equal(whichSpecies('stitch'), false);
});
});
describe('Problem 8: testNumber(number)', function() {
it('should be defined and of type function', function() {
assert(!(typeof testNumber === 'undefined'));
assert(typeof testNumber === 'function');
});
it('should return "divisible by 4" when number is divisible by 4', function() {
assert.equal(testNumber(4), 'divisible by 4');
});
it('should return "divisible by 2" when number is divisible by 2', function() {
assert.equal(testNumber(2), 'divisible by 2');
});
it('should return "divisible by 3" when number is divisible by 3', function() {
assert.equal(testNumber(3), 'divisible by 3');
});
it('should return "divisible by 5" when number is divisible by 5', function() {
assert.equal(testNumber(5), 'divisible by 5');
});
});
});
});
| 059f6eae26bfd46ba2af6875a4dbc6cfbc70669c | [
"Markdown",
"JavaScript"
] | 6 | Markdown | cjimenez113/javascript-workbook | da29ec91c68918be3bd57b7e9f2d4098ef78abfa | 92380e4619a8eaa4cecdd45f61e22951c38466e4 | |
refs/heads/master | <repo_name>julianog12/starstore<file_sep>/db/migrate/20130130121421_add_instalments_to_spree_orders.rb
class AddInstalmentsToSpreeOrders < ActiveRecord::Migration
def change
add_column :spree_orders, :instalments, :integer
end
end
<file_sep>/app/models/spree/payment_decorator.rb
Spree::Payment.class_eval do
attr_accessible :order_id, :due_date, :amount, :status
end
<file_sep>/config/initializers/spree_boleto.rb
module Spree
module Boleto
Spree::Boleto::Configuration = Spree::Boleto::Config.new
end
end
Spree::Boleto::Configuration[:parcelas] = 2
Spree::Boleto::Configuration[:banco] = "Itau"
Spree::Boleto::Configuration[:convenio] = "xxx"
Spree::Boleto::Configuration[:moeda] = "9"
Spree::Boleto::Configuration[:carteira] = "175"
Spree::Boleto::Configuration[:dias_vencimento] = 3
Spree::Boleto::Configuration[:quantidade] = 1
Spree::Boleto::Configuration[:agencia] = "xxx"
Spree::Boleto::Configuration[:conta_corrente] = "xxx"
Spree::Boleto::Configuration[:cedente] = "xxxx"
Spree::Boleto::Configuration[:documento_cedente] = "xxx"
Spree::Boleto::Configuration[:especie] = "R$"
Spree::Boleto::Configuration[:especie_documento] = "DM"
Spree::Boleto::Configuration[:instrucao1] = "Pagavel na rede bancaria ate a data de vencimento."
Spree::Boleto::Configuration[:instrucao2] = "Apos vencimento pagavel somente nas agencias do Itau."
Spree::Boleto::Configuration[:instrucao3] = "Apos vencimento cobrar multa de 2.0% e juros de 1.0% mensal."
Spree::Boleto::Configuration[:instrucao4] = "Nao receber 15 dias apos o vencimento."
Spree::Boleto::Configuration[:instrucao5] = ""
Spree::Boleto::Configuration[:instrucao6] = ""
Spree::Boleto::Configuration[:instrucao7] = ""
Spree::Boleto::Configuration[:local_pagamento] = "QUALQUER BANCO ATE O VENCIMENTO."
Spree::Boleto::Configuration[:aceite] = "N"
Spree::Boleto::Configuration[:per_page] = 10<file_sep>/README.md
starstore
=========
Loja Virtual baseada no Spree | 35238ec6d1eb02bd0d120927da463e37735a4633 | [
"Markdown",
"Ruby"
] | 4 | Ruby | julianog12/starstore | edacfaf7b7ad74a7337184696c2d19497b934e7f | 8ed87b6e66977434a01e411d2c53d1cbb3247494 | |
refs/heads/master | <file_sep>package pri.liyang.test;
import pri.liyang.service.MySQLService;
import java.sql.SQLException;
public class TestConnection {
public static void main(String[] args) throws SQLException {
System.out.println(new MySQLService().getAllTableName());
}
}
<file_sep>package pri.liyang.interfaces;
import pri.liyang.entity.FieldInfo;
import java.sql.SQLException;
import java.util.List;
import java.util.Map;
/**
* 数据库业务接口
*/
public interface DBService {
/**
* 获取当前数据库的全部表名
* @return List<String>表名
* @throws SQLException
*/
List<String> getAllTableName() throws SQLException;
/**
* 根据表名,获取该表的表结构信息
* @param tableName
* @return
* @throws SQLException
*/
List getFieldInfoListByTableName(String tableName) throws SQLException;
}
<file_sep>package pri.liyang.handler;
import pri.liyang.interfaces.ConnectHandler;
import pri.liyang.system.SystemConfig;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
/**
* MySQL的连接处理类
*/
public class MySQLConnectHandler implements ConnectHandler {
/**
* 单例Connection
*/
private static Connection connection = null;
/**
* 初始化连接
*/
static{
try {
//从配置文件获取数据库连接参数
String driver = SystemConfig.getSystemProperty("driver");
String url = SystemConfig.getSystemProperty("url");
String username = SystemConfig.getSystemProperty("username");
String password = SystemConfig.getSystemProperty("password");
//注册Driver
Class.forName(driver);
//获取Connection
connection = DriverManager.getConnection(url, username, password);
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
}
/**
* 获取连接对象
* @return Connection
*/
@Override
public Connection getConnection(){
return connection;
}
}
<file_sep>package pri.liyang.interfaces;
import java.sql.Connection;
/**
* 数据库连接处理接口
*/
public interface ConnectHandler {
/**
* 获取数据库连接
* @return
*/
Connection getConnection();
}
| ebc3b56840074babce5cd53a269da39eebc6fec5 | [
"Java"
] | 4 | Java | SynchronizedLee/DatabaseHelper | d72f0acb258d41f3e92de5a3494471248c6c43bd | 4a4401cb9d89abc8b15bb195084f611420fc84b4 | |
refs/heads/master | <repo_name>vertical-blank/ts-jsonl<file_sep>/index.ts
interface Hoge {
num: number
s: string
}
function main(): void {
const objs = jsonLinesToObjs<Hoge>('xxxxxxxxx');
console.log(objs);
objs.forEach(o => {
console.log(o.num);
console.log(o.s);
})
}
function jsonLinesToObjs<T>(v: string): T[] {
return [
JSON.parse(`{"num": 1, "s": "x"}`),
JSON.parse(`{"num": 2, "s": "y"}`),
];
}
main();
| 62c1d031b45fd15e2241a042d0629f59ce5c38dd | [
"TypeScript"
] | 1 | TypeScript | vertical-blank/ts-jsonl | cc36d5363143c9046c835b64f13ec27a53bf430c | 104c3aadec08323f4d23e18b19ac538b3313cddf | |
refs/heads/master | <file_sep>package com.swagger;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.bind.annotation.RequestMapping;
@RestController
public class CheckSwagger {
// @RequestMapping("/hi")
@PostMapping("/hi")
public String hi() {
return "hi";
}
@RequestMapping(value = "/custom", method = RequestMethod.POST)
public String custom() {
return "custom";
}
}
<file_sep>package com.swagger;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Bean
public CommandLineRunner showStatus(ApplicationContext ctx) {
return args -> {
System.out.println("CHECK STATUS OF APPLICATION CONTEXT");
String[] beanName = ctx.getBeanDefinitionNames();
for (String str : beanName) {
System.out.println("BEAN NAME:" + str);
}
};
}
}
| 36f917db0145422dcbbbf0e8eb95167bc78664cf | [
"Java"
] | 2 | Java | jeus/swagger | 50f8a7d8b0fbfd766a2ab42c78bbaa1f030a3a75 | 6a74e48c0986893397bc55c31f56e471d913e729 | |
refs/heads/master | <file_sep># Image Classification
Image classification using [TensorFlowJS](https://www.tensorflow.org/js) and Angular 10 by file upload or by webcam streaming. The [MobileNet](https://github.com/tensorflow/tfjs-models/tree/master/mobilenet) model is used to classify the image element and the streaming video element.
File upload:
* After choosing an image, the image will be loaded and the model will classify the image returning the top three predictions for that image.
Webcam Streaming:
* When the webcam is turned on, the video streaming will begin and the model will classify the HTMLVideoElement returning the top three predictions for that video element every three seconds.
<file_sep>import { Component, OnInit, ViewChild, ElementRef } from '@angular/core';
import * as mobilenet from '@tensorflow-models/mobilenet';
import * as tf from '@tensorflow/tfjs';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent implements OnInit {
title = 'image-classification';
model;
isVideo: boolean;
isImage: boolean;
imgSrc: string;
@ViewChild('img') imageEl: ElementRef;
@ViewChild('video') video: ElementRef;
predictions: Prediction[];
ngOnInit(){
console.log('loading mobilenet model...');
tf.setBackend('cpu').then(async () => {
this.model = await mobilenet.load();
});
console.log('Sucessfully loaded model');
}
streamVideo(){
this.isImage = false;
this.isVideo = true;
if (navigator.mediaDevices.getUserMedia) {
navigator.mediaDevices.getUserMedia({ video: true })
.then((stream) => {
this.video.nativeElement.srcObject = stream;
})
.catch((error) => {
console.log('Something went wrong!');
});
}
setInterval(async () => {
this.predictions = await this.model.classify(this.video.nativeElement);
await tf.nextFrame();
}, 3000);
}
turnOffWebcam(){
this.video.nativeElement.srcObject.getVideoTracks()[0].stop();
this.isVideo = false;
}
fileChange(event) {
const file = event.target.files[0];
if (file) {
const reader = new FileReader();
reader.readAsDataURL(file);
reader.onload = (res: any) => {
this.isImage = true;
this.imgSrc = res.target.result;
setTimeout(async () => {
this.predictions = await this.model.classify(this.imageEl.nativeElement);
});
};
}
}
}
export interface Prediction {
className: string;
probability: number;
} | 456a72a35e0e8d80f9d063c30836feea86a523d5 | [
"Markdown",
"TypeScript"
] | 2 | Markdown | joshg6796/image-classification | d7f4d3f9f3ad279b6a0f927198c2b1ff157ffcf1 | 4239199055efcba9c65fc273140e198295898849 | |
refs/heads/master | <file_sep># Buoydata #
A simple package for reading NOAA [global drifter program](http://www.aoml.noaa.gov/phod/dac/index.php) data.
## Download Data
Navigate to ftp://ftp.aoml.noaa.gov/pub/phod/buoydata/
Download the buoydata_*.dat files (naming conventions make it impossible to automate)
## Requirements ##
- Pandas
<file_sep>import pandas as pd
from datetime import datetime
col_names = ['id', 'mm', 'dd', 'yy',
'lat', 'lon', 'temp',
've', 'vn', 'spd',
'var_lat', 'var_lon', 'var_tmp']
def _parse_date(mm, dd, yy):
dd = float(dd)
mm = int(mm)
yy = int(yy)
day = int(dd)
hour = int(24 * (dd - day))
return datetime(yy, mm, day, hour)
def read_buoy_data(fname):
"""Read NOAA Global Drifter Program text data into pandas format.
Parameters
----------
fname : str
Returns
-------
df : datafram
"""
return pd.read_csv(fname, names=col_names, sep='\s+',
header=None, na_values=999.999,
parse_dates={'time': [1,2,3]},
date_parser=_parse_date)
<file_sep>from buoydata import buoydata
import pandas as pd
import datetime as datetime
import pytest
def test_parse_date():
mm, dd, yy = '2', '2.5', '2000'
date = buoydata._parse_date(mm,dd,yy)
assert date == datetime.datetime(2000, 2, 2, 12, 0)
@pytest.fixture(scope='session')
def datafile(tmpdir_factory):
data = """
7702986 3 8.000 1988 -1.320 274.848 25.473 999.999 999.999 999.999 0.25315E-04 0.33929E-04 0.45179E-02"""
fn = tmpdir_factory.mktemp('data').join('buoydata.dat')
fn.write(data)
return str(fn)
def test_read_buoy_data(datafile):
df = buoydata.read_buoy_data(datafile)
assert isinstance(df, pd.DataFrame)
assert len(df)==1
row = df.loc[0]
for colname in ['time', 'lat', 'lon', 'temp', 've', 'vn', 'spd',
'var_lat', 'var_lon', 'var_tmp']:
assert colname in row
assert row.id == 7702986
| a2d287ccb1ab7accc85ee5127c0cd494fdb0ddd9 | [
"Markdown",
"Python"
] | 3 | Markdown | rabernat/buoydata | 3a0686bcd8c80d08136c76c97cf601b819968df7 | 0a4495422ef99521df46462cd0465dfe1952ca66 | |
refs/heads/master | <repo_name>octoblu/meshblu-core-task-enqueue-jobs-for-subscriptions-configure-received<file_sep>/index.js
require('coffee-script/register')
module.exports = require('./src/enqueue-jobs-for-subscriptions-configure-received.coffee')
| 270260007ee515b1012a5a5df52b75f3d66d41b0 | [
"JavaScript"
] | 1 | JavaScript | octoblu/meshblu-core-task-enqueue-jobs-for-subscriptions-configure-received | c30a9952b7c54bb8967bcf66c179effa465e75c3 | a76196e4da9c87df40d0a5e035f8bb80e4d2e573 | |
refs/heads/main | <file_sep>Spyne
Lxml
py-money
<file_sep>import logging
from spyne import Application, rpc, ServiceBase, \
Integer, Decimal, Unicode
from spyne import Iterable
from spyne.protocol.soap import Soap11
from spyne.server.wsgi import WsgiApplication
from money.money import Money
from money.currency import Currency
logging.basicConfig(level=logging.DEBUG)
class ShippingService(ServiceBase):
@rpc(Decimal, Decimal, _returns=Decimal)
def compute_shipping_cost(self, distance, total_weight):
cost = Money('5', Currency.EUR) + \
(Money('0.01', Currency.EUR) + Money('0.05', Currency.EUR) * total_weight) * \
distance
return cost.amount
application = Application(
[ShippingService],
tns='info802.soap.shipping',
in_protocol=Soap11(validator='lxml'),
out_protocol=Soap11()
)
wsgi_app = WsgiApplication(application)
<file_sep>from server import wsgi_app
# App Engine by default looks for a main.py file at the root of the app
# directory with a WSGI-compatible object called app.
# This file imports the WSGI-compatible object of your Django app,
# application from mysite/wsgi.py and renames it app so it is discoverable by
# App Engine without additional configuration.
# Alternatively, you can add a custom entrypoint field in your app.yaml:
# entrypoint: gunicorn -b :$PORT mysite.wsgi
app = wsgi_app
if __name__ == '__main__':
from wsgiref.simple_server import make_server
server = make_server('127.0.0.1', 8080, wsgi_app)
print("listening to http://127.0.0.1:8080")
print("wsdl is at: http://localhost:8080/?wsdl")
server.serve_forever()
| cd16aabe42d66882a23d7c78c1052332c1366d64 | [
"Python",
"Text"
] | 3 | Text | panzergame/soap-server | e44ecfcd62493c4a620b31cf4b208af73b91c160 | 878ca3cc6284c4c4a84e6aa29514d77d31efc071 | |
refs/heads/master | <repo_name>mishraak/cmpe273_refresher_Assignment<file_sep>/javademos/src/test/java/com/cmpe273/assignment1/javademos/GenericTest.java
package com.cmpe273.assignment1.javademos;
import org.junit.Before;
import org.junit.Test;
import junit.framework.Assert;
public class GenericTest{
public GenericDemo<?> gd1, gd2;
@Before
public void initStringGeneric(){
gd1 = new GenericDemo<String>("genDemo");
gd2 = new GenericDemo<Integer>(5);
}
@Test
public void testPrintGeneric(){
Assert.assertEquals("genDemo", gd1.printArray());
Assert.assertEquals("5", gd2.printArray());
}
}
<file_sep>/javademos/src/main/java/com/cmpe273/assignment1/javademos/MultithreadingDemo.java
package com.cmpe273.assignment1.javademos;
public class MultithreadingDemo {
public int count=100;
public int decrementCount()
{
count--;
return count;
}
}<file_sep>/javademos/src/test/java/com/cmpe273/assignment1/javademos/QueueTest.java
package com.cmpe273.assignment1.javademos;
import org.junit.Before;
import org.junit.Test;
import junit.framework.Assert;
public class QueueTest {
@Before
public void initQueue(){
QueueDemo.setupQueue();
}
@Test
public void testQueue(){
Assert.assertEquals(QueueDemo.demoQueue(), "m1 m2 m3 ");
}
}
<file_sep>/javademos/src/main/java/com/cmpe273/assignment1/javademos/CollectionDemo.java
package com.cmpe273.assignment1.javademos;
import java.util.Hashtable;
public class CollectionDemo {
public Hashtable<Integer, String> ht;
public CollectionDemo(){
ht=new Hashtable<Integer, String>();
}
public int initHashMap(){
ht.put(0, "Zero");
ht.put(1, "One");
return ht.size();
}
public String updateRecord(int i, String s){
ht.put(i, s);
return ht.get(i);
}
public int deleteRecord(int i){
ht.remove(i);
return ht.size();
}
}
<file_sep>/javademos/src/test/java/com/cmpe273/assignment1/javademos/ArrayTest.java
package com.cmpe273.assignment1.javademos;
import org.junit.Before;
import org.junit.Test;
import junit.framework.Assert;
public class ArrayTest {
@Before
public void testInitArray(){
ArrayDemo.initArray();
}
@Test
public void testPrintArray(){
Assert.assertEquals(ArrayDemo.demoArray(), "0 1 4 9 16 ");
}
@Test
public void testAddToArray(){
Assert.assertEquals(6, ArrayDemo.addToArray(25));
}
}
<file_sep>/javademos/src/main/java/com/cmpe273/assignment1/javademos/Animal.java
package com.cmpe273.assignment1.javademos;
public interface Animal {
public String makeNoise();
public String callKid();
}
<file_sep>/javademos/src/test/java/com/cmpe273/assignment1/javademos/InterfaceTest.java
package com.cmpe273.assignment1.javademos;
import org.junit.Before;
import org.junit.Test;
import junit.framework.Assert;
public class InterfaceTest {
public static Animal dog, cat;
@Before
public void testInit(){
dog = new Dog();
cat = new Cat();
}
@Test
public void testCatNoise(){
Assert.assertEquals("Meow Meow!!!", cat.makeNoise());
}
@Test
public void testDogNoise(){
Assert.assertEquals("Whoo whoo!!!", dog.makeNoise());
}
@Test
public void testCatCallKid(){
Assert.assertEquals("hey kitten!!!", cat.callKid());
}
@Test
public void testDogCallKid(){
Assert.assertEquals("hey puppy!!!", dog.callKid());
}
}
| 2f2e1e270d1ec4513db4b63f6684b4126e15df55 | [
"Java"
] | 7 | Java | mishraak/cmpe273_refresher_Assignment | 2db395c647e0919fabe069424b051ae2a3321cc5 | 3ddd2376b871bc0362f2ca84654f5bb609eec914 | |
refs/heads/master | <repo_name>atla5/resume<file_sep>/README.md
# <NAME>
### Description ###
##### short #####
store and export information about my education, work experience, projects, and goals.
##### long #####
This repository is meant to act as both:
- a central hub to store the data objects about my personal and professional career in one location.
- a repeatable and simple way to generate PDF resumes without dealing with formatting hassles (allowing me to only update the json and run a command)
- a simplified way to host/serve resume info and pdf download on [my site](http://aidan-sawyer.com).
### Content ###
As a "resume", the repository is meant to store information relevant to my work
and career. Following the traditional divisions as well as those I found most
desirable to my site, the information is stored as follows:
|file|description|
|:---|:---------------|
|experience.json|jobs i've had in a number of fields|
|education.json|formal schooling i've completed|
|projects.json|software projects i've worked on over the years|
|additional.json|listing of languages, skills, interests, and links that didn't fit elsewhere|
### How to Use ###
#### Getting Started
Clone the repository or download the zip from the master branch
```
$ cd ~/your/projects/folder
$ git clone https://github.com/atla5/resume.git
```
This project runs on `python` 2.6 - 3.5 and requires `pdflatex` as a helper library.
I use/prefer macs, so the commands are in/for that. I'm sure you could get it running
on PC or linux, and if you do, please send in a ticket or Pull Request explaining
how you did.
For mac, make sure you have homebrew package manager installed:
```bash
$ /bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
```
And use it to install the `basictex` [brew cask](https://brew.sh/).
```bash
$ brew install basictex --cask
```
#### Running the Builder
The main script for this repository, responsible for reading the json,
populating the new `.tex` file, and outputing the actual `.pdf` at the end
is in `src/build_resume.pdf`:
```bash
$ python src/build_resume.py
```
Once you run it, look in the `./build` folder to find the following main outputs:
- `Resume_NAME.pdf`
- `CoverLetter_NAME`
- `References_NAME.pdf`
#### Rolling Your Own
You probably don't just want to build _my_ resume, so if you'd like to create one
that looks like it but contains your own information, simply edit the `.json` files
in the `data/` directory.
If you don't like how I laid out my `json` schemas and want to fiddle with how the information
is stored, look into editing the `src/update_values_helpers.py` methods.
More extensive edits can be made to the _content_ of the resume by editing `build/resume.tex`
directly. The `.tex` is similar to most other markup once you get the hang of it, and
doesn't take you long to learn.
If you'd like to make changes to the _styling_ of the resume, you're looking at changing
the `build/resume.cls` file, and more power to you. I've yet to wrestle that beast, and
will not be able to provide you much assistance (at least yet).
<file_sep>/src/update_values_helpers.py
# author: <NAME> (atla5)
# date_created: 2017-08-12
# license: MIT
# purpose: customize dict_values according to passed values
import logging
logger = logging.getLogger(__name__)
months = ["Jan", "Feb", "March", "April", "May", "June", "July", "Aug", "Sept", "Oct", "Nov", "Dec"]
months_full = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]
def humanize_date(yyyy_mm, formalize=False):
output = yyyy_mm
try:
if '-' not in yyyy_mm:
return yyyy_mm
else:
tokens = yyyy_mm.split('-')
year = tokens[0]
month = int(tokens[1])
if 0 < month <= 12:
str_month = months_full[month-1] if formalize else months[month-1]
output = "{} {}".format(str_month, year)
else:
logger.warning("Invalid month: {}\n".format(yyyy_mm))
except IndexError:
logger.warning("Improperly formatted date: {}\n".format(yyyy_mm))
finally:
return output
def humanize_list(ls):
return ", ".join(str(s) for s in ls)
def generate_about(dict_values, about):
contact = about['contact']
accounts = about['accounts']
highlights = about['overview']
dict_values.update({
"FULL~NAME": about['name'],
"OBJECTIVE": about['objective'],
"EMAIL": contact['email'] if contact['email'] else "",
"PHONE": contact['phone'] if contact['phone'] else "",
"GITHUB": accounts['github'],
"WEBSITE": about['url'].replace('http://', ''),
"HIGHLIGHT~1": highlights[0],
"HIGHLIGHT~2": highlights[1]
})
def generate_school_info(dict_values, school, id=1):
logging.debug("updating school values...")
prefix = "S{}~".format(id)
school_notes = school['notes']
dict_values.update({
prefix + "NAME": school['school_name'],
prefix + "DEGREE": school['degree'],
prefix + "TIME~START": humanize_date(school['time_start']),
prefix + "TIME~END": humanize_date(school['time_end']),
prefix + "SUMMARY": school['summary'] if 'summary' in school else school_notes[0],
prefix + "NOTE~1": school_notes[0],
prefix + "NOTE~2": school_notes[1] if len(school_notes) >= 2 else ""
})
def generate_work_experience(dict_values, work, pre="WA", id=1):
logging.debug("updating work experience values for work '{}'".format(id))
prefix = "{}{}~".format(pre,id) # e.g. 'WA1~', 'WB2~'
responsibilities = work['responsibilities']
num_responsibilities = len(responsibilities)
dict_values.update({
prefix + "NAME": work['company_name'],
prefix + "POSITION": work['position'],
prefix + "TIME~START": humanize_date(work['time_start']),
prefix + "TIME~END": humanize_date(work['time_end']) if 'time_end' in work else "Present",
prefix + "ADVISOR~NAME": work['advisor_name'],
prefix + "ADVISOR~POSITION": work['advisor_position'],
prefix + "ADVISOR~CONTACT": work['advisor_contact'],
prefix + "RESPONSIBILITY~1": responsibilities[0] if num_responsibilities >= 1 else work['summary_short'],
prefix + "RESPONSIBILITY~2": responsibilities[1] if num_responsibilities >= 2 else "",
prefix + "RESPONSIBILITY~3": responsibilities[2] if num_responsibilities >= 3 else "",
prefix + "SUMMARY": work['summary_short'] if 'summary_short' in work else ""
})
def generate_reference(dict_values, reference, id=1):
logging.debug("updating reference '{}'".format(id))
prefix = "R{}~".format(id)
contact = reference['email']
if 'phone' in reference and reference['phone']:
contact += " - {}".format(reference['phone'])
dict_values.update({
prefix + "NAME": reference['name'],
prefix + "CONTACT": contact,
prefix + "POSITION": reference['position'],
prefix + "DATE~START": humanize_date(reference['date_start']),
prefix + "DATE~END": humanize_date(reference['date_end']) if 'date_end' in reference else "Present",
prefix + "RELATIONSHIP": reference['relationship'],
prefix + "IMPORTANCE": reference['importance'],
})
def generate_project(dict_values, project, id=1):
logging.debug("updating project info for project '{}'".format(id))
prefix = "P{}~".format(id)
dict_values.update({
prefix+"NAME": project['name'],
prefix+"DESCRIPTION": project['description_short']
})
def generate_certificate(dict_values, certificate, id=1):
logging.debug("updating project info for certificate '{}'".format(id))
prefix = "C{}~".format(str(id))
dict_values.update({
prefix+"CODE": certificate['name'],
prefix+"DESCRIPTION": certificate['description']
})
def generate_language_entry(dict_values, level, languages, id=1):
logging.debug("updating language entry for level '{}'".format(level))
suffix = "~{}".format(id)
dict_values.update({
"LEVEL" + suffix: level if languages else "",
"LANGUAGES" + suffix: humanize_list([lang['name'] for lang in languages] if languages else "")
})
def generate_languages(dict_values, languages):
# establish name for proficiencies
lvl_1 = "Intermediate"
lvl_2 = "Functional"
lvl_3 = "Novice"
# sort languages into lists based on proficiency
ls_intermediate = []
ls_functional = []
ls_limited = []
for language in languages:
if language['proficiency'] == lvl_1:
ls_intermediate.append(language)
elif language['proficiency'] == lvl_2:
ls_functional.append(language)
else:
ls_limited.append(language)
# update dict_values with each grouping of languages
generate_language_entry(dict_values, lvl_1, ls_intermediate, 1)
generate_language_entry(dict_values, lvl_2, ls_functional, 2)
generate_language_entry(dict_values, lvl_3, ls_limited, 3)
<file_sep>/src/build_resume.py
# author: <NAME> (atla5)
# date_created: 2017-08-12
# license: MIT
# purpose: build custom resume from LaTeX template and json
import json, time, logging
from os import path, getcwd, system, chdir
from sys import stdout
from shutil import copyfile
from subprocess import check_call, STDOUT, DEVNULL
from update_values_helpers import *
logging.basicConfig(stream=stdout, level=logging.INFO)
logger = logging.getLogger("build_resume")
# set absolute paths for 'build/' and 'data/' directories
src_dir = path.abspath(path.dirname(__file__))
build_dir = path.abspath(path.join(src_dir, "../build"))
data_dir = path.abspath(path.join(src_dir, "../data"))
def get_json_from_data_file(filename):
json_to_return = {}
try:
data_file = path.join(data_dir, filename)
json_to_return = json.load(open(data_file))
except FileNotFoundError:
logger.error("Error loading file: {}".format(filename), exc_info=True)
finally:
return json_to_return
def sanitize_latex_syntax(line):
return line.replace("#", "\#")
def update_shared_values(dict_values):
logger.debug("adding header, date data to 'dict_values'")
# about me
about = get_json_from_data_file('about.json')
generate_about(dict_values, about)
# date created
dict_values.update({
"DATE~CREATED": time.strftime("%Y-%m-%d")
})
def update_resume_values(dict_values):
logger.debug("adding resume values data to 'dict_values'")
filter_a = "craft"
filter_b = "software"
# education
educations = get_json_from_data_file('education.json')
filtered = [ed for ed in educations if filter_a in ed['tags'] or filter_b in ed['tags']]
generate_school_info(dict_values, filtered[0], 1)
if filtered[1]:
generate_school_info(dict_values, filtered[1], 2)
# work experiences A (e.g. "craft")
experiences = get_json_from_data_file('experience.json')
work_xp = [xp for xp in experiences if filter_a in xp['tags']]
for i, work_experience in enumerate(work_xp, start=1):
generate_work_experience(dict_values, work_experience, pre="WA", id=i)
# work experiences B (e.g. "software")
pro_xp = [xp for xp in experiences if filter_b in xp['tags']]
for i, work_experience in enumerate(pro_xp[:3], start=1):
generate_work_experience(dict_values, work_experience, "WB", i)
# projects
projects = get_json_from_data_file('projects.json')
for i, project in enumerate(projects[:3], start=1):
generate_project(dict_values, project, i)
# languages
additional = get_json_from_data_file('additional.json')
languages = additional['languages']
generate_languages(dict_values, languages)
# certificates
certificates = additional['certifications']
for i, certificate in enumerate(certificates[:4], start=1):
generate_certificate(dict_values, certificate, i)
def update_references_values(dict_values):
logger.debug("adding references data to 'dict_values'")
references = get_json_from_data_file('references.json')
for i, project in enumerate(references[:3], start=1):
generate_reference(dict_values, project, i)
def generate_new_tex_file_with_values(values, input_template, output_filename):
logger.debug("generating new tex file '{}' using input template '{}'".format(output_filename, input_template))
# copy .tex template into a new 'output_filename.tex'
copyfile(input_template, output_filename)
# use `dict_values` to replace placeholders in template with real values in the new one
resume_template = open(input_template, 'r')
output_tex = open(output_filename, 'w')
for line in resume_template:
for key in values:
line = line.replace(key, values[key])
output_tex.write(sanitize_latex_syntax(line))
# close files
resume_template.close()
output_tex.close()
def generate_pdf_from_tex_template(output_tex_filename):
logger.debug("generating pdf from tex file '{}'".format(output_tex_filename))
chdir(build_dir)
# export filename.tex into a pdf
check_call(['pdflatex', '-interaction=nonstopmode', output_tex_filename], stdout=DEVNULL, stderr=STDOUT)
logger.info("pdf created at {}".format(output_tex_filename.replace('.tex','.pdf')))
def build_resume():
logger.info("\n\nbuilding resume...")
# create and update value dictionary from json files
dict_values = {}
update_shared_values(dict_values)
update_resume_values(dict_values)
# manage/generate filenames and paths
tex_template_filepath = path.join(build_dir, "resume.tex")
last_name = dict_values['FULL~NAME'].split()[-1]
filename = "Resume{}".format("_"+last_name if last_name else "")
tex_new_filepath = path.join(build_dir, filename + ".tex")
# use values to generate a pdf
generate_new_tex_file_with_values(dict_values, tex_template_filepath, tex_new_filepath)
generate_pdf_from_tex_template(tex_new_filepath)
def build_references():
logger.info("\n\nbuilding references...")
# create and update value dictionary from json files
dict_values = {}
update_shared_values(dict_values)
update_references_values(dict_values)
# manage/generate filenames and paths
tex_template_filepath = path.join(build_dir, "references.tex")
last_name = dict_values['FULL~NAME'].split()[-1]
filename = "References{}".format("_" + last_name if last_name else "")
tex_new_filepath = path.join(build_dir, filename + ".tex")
# use values to generate a pdf
generate_new_tex_file_with_values(dict_values, tex_template_filepath, tex_new_filepath)
generate_pdf_from_tex_template(tex_new_filepath)
def build_cover_letter():
logger.info("\n\nbuilding cover letter...")
dict_values = {}
update_shared_values(dict_values)
with open(path.join(data_dir, "coverletter.txt"),'r') as cover_letter_text:
cl_text = ""
for line in cover_letter_text:
cl_text += line.replace("’", "'").replace("‘","'")
dict_values.update({
"CL~TEXT": cl_text,
"FORMAL~DATE": humanize_date(dict_values["DATE~CREATED"], formalize=True)
})
# manage/generate filenames and paths
tex_template_filepath = path.join(build_dir, "coverletter.tex")
last_name = dict_values['FULL~NAME'].split()[-1]
filename = "CoverLetter{}".format("_" + last_name if last_name else "")
tex_new_filepath = path.join(build_dir, filename + ".tex")
# use values to generate a pdf
generate_new_tex_file_with_values(dict_values, tex_template_filepath, tex_new_filepath)
generate_pdf_from_tex_template(tex_new_filepath)
def clean_up():
system("rm *.aux")
system("rm *.fls")
system("rm *.aux")
system("rm *.gz")
system("rm *latexmk")
if __name__ == "__main__":
build_resume()
build_references()
build_cover_letter()
clean_up()
<file_sep>/src/test_build.py
import unittest
import os, json, csv
from update_values_helpers import humanize_date, humanize_list
from build_resume import build_dir, data_dir, build_resume, build_references
def get_time_created_or_zero(filename):
return os.path.getctime(filename) if os.path.exists(filename) else 0
class TestBuild(unittest.TestCase):
def test_humanize_date(self):
self.assertEqual("Jan 2017", humanize_date("2017-01"))
self.assertEqual("2017-13", humanize_date("2017-13"))
self.assertEqual("2017-00", humanize_date("2017-00"))
def test_humanize_list(self):
self.assertEqual("one fish, two fish, red fish, blue fish", humanize_list(["one fish", "two fish", "red fish", "blue fish"]))
self.assertEqual("one fish, two fish", humanize_list(["one fish", "two fish"]))
self.assertEqual("one fish", humanize_list(["one fish"]))
self.assertEqual("", humanize_list([""]))
self.assertEqual("1, 2, 3, 4", humanize_list([1, 2, 3, "4"]))
def test_valid_json_data(self):
for filename in os.listdir(data_dir):
if filename.endswith('.json'):
try:
with open(os.path.join(data_dir, filename), 'r') as file:
json.loads(file.read())
except ValueError:
self.fail("linting error found in {}".format(filename))
def test_valid_csv_data(self):
for filename in os.listdir(data_dir):
if filename.endswith('.csv'):
try:
with open(os.path.join(data_dir, filename), 'r') as csvfile:
for row in csv.reader(csvfile, delimiter=','):
if not bool(row) or not isinstance(row, list) or not all(isinstance(elem, str) for elem in row):
self.fail("error rendering csv file '{}' into list of strings.".format(filename))
except Exception:
self.fail("error reading csv file: {}".format(filename))
def build_test_helper(self, filename_prefix, build_function):
# get the 'created' timestamp any existing output files were created, defaulting to zero
output_tex_timestamp_before = get_time_created_or_zero("{}.tex".format(filename_prefix))
output_pdf_timestamp_before = get_time_created_or_zero("{}.pdf".format(filename_prefix))
try:
build_function()
except Exception:
self.fail("Exception occurred while attempting to build {} output".format(filename_prefix))
# get the 'created' timestamp of our fresh output files
output_tex_timestamp_after = get_time_created_or_zero("{}.tex".format(filename_prefix))
output_pdf_timestamp_after = get_time_created_or_zero("{}.pdf".format(filename_prefix))
# ensure that build_resume() effectively created both files
if output_tex_timestamp_after != 0:
self.assertGreater(output_tex_timestamp_after, output_tex_timestamp_before)
else:
self.fail("Error Creating {}.tex".format(filename_prefix))
if output_pdf_timestamp_after != 0:
self.assertGreater(output_pdf_timestamp_after, output_pdf_timestamp_before)
else:
self.fail("Error creating {}.pdf".format(filename_prefix))
def test_build_resume(self):
self.build_test_helper("Resume_Sawyer", build_resume) # todo: make this filename prefix generic
def test_build_references(self):
self.build_test_helper("References_Sawyer", build_references) # todo: make this filename prefix generic
| b5a32f11a57e8cbcd398cd580b2be6904e6cede4 | [
"Markdown",
"Python"
] | 4 | Markdown | atla5/resume | 8ec0306af617446cb5cc76ca12be426152d15573 | 7e2010429912b24f90d40a3b04926ea5825cf387 | |
refs/heads/master | <repo_name>magalyhernandez/Ingenieria-de-Software-2018<file_sep>/Patrones/index.php
<!DOCTYPE html>
<html lang="es" dir="ltr">
<head>
<?php include "secciones/head.php"?>
</head>
<body>
<?php $menu="secciones/menu.php" ?>
<?php include "secciones/header.php" ?>
<?php include "secciones/patrones.php" ?>
<?php include "secciones/codigo.php" ?>
<?php include "secciones/informacion.php"?>
<?php include "secciones/wikis.php"?>
<?php include "secciones/footer.php"?>
<?php include "secciones/modalsPatrones.php" ?>
<?php include "secciones/modalsCodigo.php" ?>
<?php include "secciones/scripts.php"?>
</body>
</html>
| c3db8860135e9a4e6f34c82e7fdca9a072d4c50c | [
"PHP"
] | 1 | PHP | magalyhernandez/Ingenieria-de-Software-2018 | 2b84bb19e1dc2629069d4975a7cd9d7e86c7b6b7 | c7abd8d1c586d6db072dff5c5db63ee61f152c1d | |
refs/heads/master | <file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyAttack : MonoBehaviour
{
// Start is called before the first frame update
Animator animator;
public Transform attackPoint;
public float attackRange;
public LayerMask playerLayer;
new Rigidbody rigidbody;
public AnimationClip attackAnimation;
float nextAttackTime = 0f;
void Start()
{
rigidbody = GetComponent<Rigidbody>();
animator = GetComponent<Animator>();
}
// Update is called once per frame
public void Attack(){
rigidbody.velocity = Vector3.zero;//stop enemy moving
Collider[] players = Physics.OverlapSphere(attackPoint.position, attackRange, playerLayer);
if (Time.time >= nextAttackTime)
{
// call attack animation
animator.SetTrigger("Attack");
Debug.Log("enemy attack");
foreach (var player in players)
{
player.GetComponentInParent<Player>().TakeDamaged(1);
}
nextAttackTime = Time.time + attackAnimation.length;
}
}
private void OnDrawGizmosSelected() {
Gizmos.DrawSphere(attackPoint.position, attackRange);
}
}
<file_sep>using UnityEngine;
using UnityEngine.SceneManagement;
public class GameManager : MonoBehaviour
{
public bool isGameEnded = false;
GameObject[] enemies;
Animator gameOverAnim;
private void Start() {
gameOverAnim = GameObject.Find("TransitionScreen").transform.GetComponent<Animator>();
}
public void GameOver(){
gameOverAnim.SetTrigger("GameOver");//trigger fade out animation)
}
public void Winning(){
gameOverAnim.SetTrigger("Winning");//trigger fade out animation)
}
public void LoadGameOverScene(){//use when gameoverAnim is ended
SceneManager.LoadScene("GameOver");
}
public void LoadWinningScene(){//use when gameoverAnim is ended
SceneManager.LoadScene("Winning");
}
private void Update() {
enemies = GameObject.FindGameObjectsWithTag("Enemy");
if(enemies.Length == 0){
Winning();//if enemies are 0 game over
}
}
}<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class HealthBarBehaviour : MonoBehaviour
{
public Color low;
public Color high;
public Vector3 offset;
public bool isEnemyHealthBar = false;
public Slider slider;
public void SetMaxHealth(float maxhealth){
slider.maxValue = maxhealth;
slider.value = maxhealth;
}
public void SetHealth(float health){
// slider.gameObject.SetActive(health < maxhealth);//show healthbar when enemy healthbar is not full
slider.value = health;
}
// Update is called once per frame
void Update()
{
if (isEnemyHealthBar)
{//if this health used by enemy object
Vector3 position = Camera.main.WorldToScreenPoint(transform.parent.position + offset);
slider.transform.position = position;
}
}
public void SetHealthBarActivated(bool condition){//display health bar depend on some condition
slider.gameObject.SetActive(condition);
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerCombat : MonoBehaviour
{
// Start is called before the first frame update
public Transform Sword;
public Animator swordAnimator;
public Transform trailRenderer;
public LayerMask enemyLayer;
public Transform attackPoint;
public float attackRange;
void Start()
{
// Sword = gameObject.transform.GetChild(0).gameObject.transform.GetChild(0).gameObject;
Sword.GetComponent<SpriteRenderer>().enabled = false;
}
// Update is called once per frame
void Update()
{
if (Input.GetAxis("Fire1") > 0)
{
Sword.GetComponent<SpriteRenderer>().enabled = true;
trailRenderer.GetComponent<TrailRenderer>().enabled = true;
Attack();
}
}
public void EnableRenderer(){
Sword.GetComponent<SpriteRenderer>().enabled = false;
trailRenderer.GetComponent<TrailRenderer>().enabled = false;
}
void Attack(){
swordAnimator.SetTrigger("Attack");
Collider[] enemies = Physics.OverlapSphere(attackPoint.position, attackRange, enemyLayer);
foreach (var enemy in enemies)
{
Debug.Log(enemy.name);
enemy.GetComponent<Enemy>().TakeDamaged(5);
}
}
private void OnDrawGizmosSelected() {
Gizmos.DrawSphere(attackPoint.position, attackRange);
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed;
public Rigidbody playerRb;
public Vector3 movement;
public GameObject playerSprite;
GameObject playerpointLight;
bool isFlipped = false;
bool IsGrounded = false;//boolean when player collide with ground
Vector3 jumpVector = new Vector3(0,1,0);
Vector3 newPlayerLocalScale;//reference for new player localscale
public float jumpForce;
Animator animator;//animator for player
public Animator camAnim;
public ParticleSystem landingParticle;
public ParticleSystem runParticle;
// Start is called before the first frame update
void Start()
{
animator = transform.GetChild(0).GetComponent<Animator>();
playerRb = GetComponent<Rigidbody>();
newPlayerLocalScale = new Vector3(1,1,1);
}
// Update is called once per frame
void Update()
{
movement.x = Input.GetAxis("Horizontal");
movement.z = Input.GetAxis("Vertical");
RotateDirection(movement.x);
movement*= speed;
//intantiate run animation
RunAnimate(movement.x, movement.z);
if (Input.GetKeyDown(KeyCode.Space) && IsGrounded)
{
IsGrounded = false;//change isGrounded value to false then jump
Jump();
}
}
void RunAnimate(float movementX, float movementZ){
int speed = 0;//speed variable to instantiate run animation
var movementXAbs = Mathf.Abs(movementX);//get absolute value from x axis
var movementZAbs = Mathf.Abs(movementZ);//get absolute value from z axis
speed = movementXAbs > 0 || movementZAbs > 0? 1: -1; // if movement X or Z abs greeater than 0, then assigned 1 to speed variable, and otherwise assign -
animator.SetFloat("Run",speed);
}
private void FixedUpdate() {
playerRb.velocity = new Vector3(movement.x, playerRb.velocity.y, movement.z);
}
private void RotateDirection(float xAxis) {
if (xAxis < 0 && !isFlipped)
{//change variable reference value base on player x axis value(right or left move)
isFlipped = true;
newPlayerLocalScale = new Vector3(-1,1,1);
}
if(xAxis > 0 && isFlipped){
isFlipped = false;
newPlayerLocalScale = new Vector3(1,1,1);
}
FlipPlayerObject(newPlayerLocalScale);
}
private void FlipPlayerObject(Vector3 newLocalScale){
playerSprite.transform.localScale = newLocalScale;
}
private void OnCollisionStay(Collision collider) {
if (collider.gameObject.tag == "Ground")
{
if(!runParticle.isEmitting){//if run particle is not emmiting play particle
runParticle.Play();
}
IsGrounded = true;
}
}
private void OnCollisionExit(Collision collider) {
if (collider.gameObject.tag == "Ground")
{//stop particle when player jump on ground
if(runParticle.isEmitting){//if run particle is emmiting stop particle
runParticle.Stop();
}
IsGrounded = false;
}
}
private void OnCollisionEnter(Collision collider) {
if (collider.gameObject.tag == "Ground")
{
if (!landingParticle.isEmitting)//check wheter landing particle is not emiting
{
//camera shake effect
Camera.main.GetComponent<FollowPlayer>().Shake(1f, 10);
landingParticle.Play();//play landing particle
}
}
}
private void Jump(){
playerRb.AddForce(Vector3.up*jumpForce, ForceMode.Impulse);
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyDetection : MonoBehaviour
{
Transform PlayerObject;
float distanceToPlayer;
new Rigidbody rigidbody;
Animator animator;
float enemyAttackRange;
public float enemyChaseRange = 2.4f;
public float moveSpeed = 1f;
bool isFlipped = false;
Vector3 newLocalScale;
// Start is called before the first frame update
void Start()
{
newLocalScale = GetComponent<Transform>().localScale;
enemyAttackRange = GetComponent<EnemyAttack>().attackRange;
animator = GetComponent<Animator>();
rigidbody = GetComponent<Rigidbody>();
PlayerObject = GameObject.Find("PlayerObject").transform.GetChild(0);
}
// Update is called once per frame
void Update()
{
distanceToPlayer = Vector3.Distance(PlayerObject.transform.position, transform.position);//get distance from player to enemy
// Debug.Log("distance between "+transform.name+" and player is"+distanceToPlayer);
if(distanceToPlayer < enemyChaseRange){
ChasePlayer();
if(distanceToPlayer < enemyAttackRange){
StopChase();
GetComponent<EnemyAttack>().Attack();
}
}else{
StopChase();
}
}
private void FlipPlayerObject(Vector3 newLocalScale){
transform.localScale = newLocalScale;
}
void ChasePlayer(){
// Debug.Log("im chasing you");
var newpos = Vector3.MoveTowards(rigidbody.position, PlayerObject.position, moveSpeed*Time.deltaTime);
// change enemy faced
if (PlayerObject.transform.position.x > transform.position.x && !isFlipped){
isFlipped = true;
newLocalScale = new Vector3(-newLocalScale.x,newLocalScale.y,newLocalScale.z);
}
if(PlayerObject.transform.position.x < transform.position.x && isFlipped){
isFlipped = false;
newLocalScale = new Vector3(Mathf.Abs(newLocalScale.x),newLocalScale.y,newLocalScale.z);
}
FlipPlayerObject(newLocalScale);//asign new local scale
//start running animation
animator.SetFloat("Running", 1);
rigidbody.MovePosition(newpos);
}
void StopChase(){
rigidbody.velocity = Vector3.zero;
//stop running animation
animator.SetFloat("Running", -1);
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class Enemy : MonoBehaviour
{
// Start is called before the first frame update
public float maxhealth;
float currentHealth;
new Rigidbody rigidbody;
public ParticleSystem walkParticle;
public ParticleSystem landingParticle;
public ParticleSystem dieParticle;
public Animator animator;
public HealthBarBehaviour healthBar;
public AudioSource bloodSfx;
void Start()
{
rigidbody = GetComponent<Rigidbody>();
currentHealth = maxhealth;
//set halthbar to enemy
healthBar.SetMaxHealth(maxhealth);
}
private void Update() {
healthBar.SetHealthBarActivated(currentHealth < maxhealth);
}
private void FixedUpdate() {
if (transform.position.y < -3)
{
Die();
}
}
public void TakeDamaged(int damage){
animator.SetTrigger("Attacked");
//Decrease enemy health
currentHealth -= damage;
//set healthbar value
healthBar.SetHealth(currentHealth);
//knock back enemy
rigidbody.AddForce(Vector3.up*1f, ForceMode.Impulse);
if(currentHealth <= 0){
if(!dieParticle.isEmitting){
dieParticle.Play();//start blood particle
bloodSfx.Play();//play sfx
}
Die();
}
}
void Die(){
Debug.Log("die");
Destroy(gameObject, dieParticle.main.duration);
}
private void OnCollisionStay(Collision other) {
if(other.gameObject.tag == "Ground"){
if(!walkParticle.isEmitting){
//if particle not emmiting play particle
walkParticle.Play();
}
}
}
private void OnCollisionExit(Collision other) {
if(other.gameObject.tag == "Ground"){
if(walkParticle.isEmitting){
walkParticle.Stop();//stop walk particle
}
if(!landingParticle.isEmitting){
landingParticle.Play();//play landing particle
}
}
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Player : MonoBehaviour
{
// Start is called before the first frame update
public float maxhealth = 100;
float currentHealth;
new Rigidbody rigidbody;
public HealthBarBehaviour healthBar;
public Animator animator;
public AudioSource bgMusic;
void Start()
{
rigidbody = GetComponent<Rigidbody>();
currentHealth = maxhealth;
healthBar.SetMaxHealth(maxhealth);
}
private void FixedUpdate() {
if (transform.position.y < -3)
{
Die();
}
}
public void tes(){
Debug.Log("kasjdlsad");
}
public void TakeDamaged(int damage){
animator.SetTrigger("Damaged");
//Decrease player health
currentHealth -= damage;
//set healthbar value
healthBar.SetHealth(currentHealth);
//knock back player
// rigidbody.AddForce(new Vector3(rigidbody.position.x, 0, rigidbody.position.z));
rigidbody.AddForce(Vector3.up*2f, ForceMode.Impulse);
if(currentHealth <= 0){
Die();
}
}
void Die(){
Debug.Log("die");
bgMusic.Stop();
Destroy(gameObject);
GameObject.Find("TransitionScreen").transform.GetComponent<GameManager>().GameOver();//call gameover fucntion from gamemanager script
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GrassMoving : MonoBehaviour
{
// Start is called before the first frame update
Animator animator;
void Start()
{
animator = GetComponent<Animator>();
}
// Update is called once per frame
// private void OnTriggerEnter(Collider collider) {
// if (collider.gameObject.tag == "Player"){
// //when player collide with grass trigger "forced" grass animation
// animator.SetTrigger("TouchedPlayer");
// }
// }
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FollowPlayer : MonoBehaviour
{
public Transform playerTransform;
new Camera camera;
public Vector3 offSet;
// Start is called before the first frame update
void Start()
{
camera = Camera.main;
}
// Update is called once per frame
void Update()
{
camera.transform.position = playerTransform.position + offSet;
}
public IEnumerator Shake(float duration, float magnitude){
Vector3 originalPos = transform.localPosition;
float elapse = 0.5f;
while (elapse <duration)
{
float x = Random.Range(-1,1) * magnitude;
float y = Random.Range(-1,1) * magnitude;
transform.localPosition = new Vector3(x,y, originalPos.z);
elapse += Time.deltaTime;//increment sec time
yield return null;
}
transform.localPosition = originalPos;
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class DecorationBehavior : MonoBehaviour
{
// Start is called before the first frame update
Color transparent;
Color normal;
void Start()
{
transparent = GetComponent<SpriteRenderer>().color;
normal = GetComponent<SpriteRenderer>().color;
transparent.a = 0.5f;
normal.a = 1f;
}
private void OnTriggerStay(Collider collider) {
if (collider.gameObject.tag == "Player")
{
GetComponent<SpriteRenderer>().color = transparent;
}
}
private void OnTriggerExit(Collider collider) {
if (collider.gameObject.tag == "Player")
{
GetComponent<SpriteRenderer>().color = normal;
}
}
// Update is called once per frame
void Update()
{
}
}
| ec9a786078877d4cfffe6a6fdbc8a2f7c63a3320 | [
"C#"
] | 11 | C# | SeptAlfauzan/unfinished-world | bfe562ffa136f341c9b5c8e9f9ea285ef1cd4c6e | aa8401ffca9f655bc85cf09f2b18d29f99849851 | |
refs/heads/master | <repo_name>camkaley/Ethereum-Price-Tracker<file_sep>/ethereum.py
# -*- coding: utf-8 -*-
"""
Created on Mon May 29 15:41:13 2017
@author: camka
"""
from urllib.request import urlopen
import json
import time
import os
a = 0
myeth = 10.5
moneypaid = 5200
moneypaidusd = 4077.32
data = open("ethereum.txt", "r")
datalines = data.readlines()
firstprice = datalines[0]
oldprice = datalines[len(datalines) - 1]
data.close()
firstprice = firstprice.split(":")[0]
oldprice = oldprice.split(":")[0]
pricearray = []
datearray = []
while 1 == 1:
try:
response = urlopen('https://api.coinbase.com/v2/exchange-rates')
html = response.read()
parsed_json = json.loads(html)
oneusd = parsed_json['data']['rates']['ETH']
oneaud = parsed_json['data']['rates']['AUD']
ethusd = 1 / float(oneusd)
audeth = ethusd * float(oneaud)
if (a == 0):
data = open("ethereum.txt", "a")
data.write(str(audeth) + ":" + time.strftime("%d/%m/%Y") + "\n")
data.close();
a = 1
for line in datalines:
line = line.split(":")
price = line[0]
date = line[1]
date = date.replace("/", "")
pricearray.append(price)
datearray.append(date)
change = round(((audeth / float(oldprice)) * 100 - 100), 2)
totalchange = round(((audeth / float(firstprice)) * 100 - 100), 2)
profitaud = (myeth * audeth) - moneypaid
profitusd = (myeth * ethusd) - moneypaidusd
print("Ethereum Price AUD: $" + str(round((audeth), 2 )))
print("Ethereum Price USD: $" + str(round((ethusd), 2 )))
print("Percent Changed Since Last Round: " + str(change) + "%")
print("Percent Changed Overall: " + str(totalchange) + "%")
print("Profit: ~ $" + str(round((profitaud), 2 )) + " AUD / $" + str(round((profitusd), 2 )) + " USD")
time.sleep(60)
os.system('cls')
except:
print("Error connection to API, retrying in 3 seconds...")
time.sleep(3)
<file_sep>/README.md
# Ethereum-Price-Tracker
A simple python script using an API from coinbase (https://api.coinbase.com/v2/exchange-rates). Simply gets the price of 1 ETH in and converts it to its price in AUD, showing how much it has changed in price since the last time the program was run and the profit determined by what is set in the program settings.
| 201e2edf57bbe5c82243b1703c7c5ced2f2533f8 | [
"Markdown",
"Python"
] | 2 | Python | camkaley/Ethereum-Price-Tracker | 1fd5ca4337847066dc179442f55c31ddca81121c | 3cb941c50442bf8f2bc8151990fdd68a4ea4671a | |
refs/heads/master | <repo_name>isliulin/TimeFrequency<file_sep>/MCPU/ext_ctx.h
#ifndef __EXT_CTX__
#define __EXT_CTX__
#include "ext_type.h"
#define EXT_DRV_NUM 2
#define EXT_MGR_NUM 6
#define EXT_OUT_NUM 30
#define EXT_GRP_NUM 3
#define EXT_1_OUT_LOWER 23
#define EXT_1_OUT_UPPER 32
#define EXT_1_PWR1 35
#define EXT_1_PWR2 36
#define EXT_1_MGR_PRI 37
#define EXT_1_MGR_RSV 38
#define EXT_1_OUT_OFFSET (EXT_1_OUT_LOWER)
#define EXT_2_OUT_LOWER 39
#define EXT_2_OUT_UPPER 48
#define EXT_2_PWR1 51
#define EXT_2_PWR2 52
#define EXT_2_MGR_PRI 53
#define EXT_2_MGR_RSV 54
#define EXT_2_OUT_OFFSET (EXT_2_OUT_LOWER - 10)
#define EXT_3_OUT_LOWER 55
#define EXT_3_OUT_UPPER 66//58,59不使用
#define EXT_3_PWR1 67
#define EXT_3_PWR2 68
#define EXT_3_MGR_PRI 69
#define EXT_3_MGR_RSV 70
#define EXT_3_OUT_OFFSET (EXT_3_OUT_LOWER - 20)
#define EXT_SAVE_PATH "/var/p300file"
#define EXT_SAVE_ADDR 0x1F0000
enum
{
EXT_NONE = 'O',
EXT_PWR = 'M',
EXT_MGR = 'f',
EXT_OUT16 = 'J',
EXT_OUT32 = 'a',
EXT_DRV = 'd',
EXT_OUT32S = 'h',
EXT_OUT16S = 'i'
};
struct extdrv
{
/*
1 主DRV
2 备DRV
3 通信故障
4 未连接
*/
u8_t drvPR[EXT_GRP_NUM];
/*
DRV和MGR之间的连接状态,1个字节
1 未连接
0 已连接
0100Y3Y2Y1Y0
Y0-Y2,分别表示DRV和MGR(1-3)之间的连接状态
*/
u8_t drvAlm;
/*版本信息*/
u8_t drvVer[23];
};
struct extmgr
{
/*
1 主MGR
2 备MGR
*/
u8_t mgrPR;
/*
MGR和输出板卡之间的通讯告警,3个字节
1 告警
0 正常
0100Y3Y2Y1Y0 0100Y7Y6Y5Y4 0100Y11Y10Y9Y8
Y0-Y9,分别表示MGR和输出板卡(1-10)之间的通讯告警
Y10 表示PWR1告警
Y11 表示PWR2告警
Y12 1槽位拔盘告警
Y13 2槽位拔盘告警
Y14 3槽位拔盘告警
Y15 4槽位拔盘告警
Y16 5槽位拔盘告警
Y17 6槽位拔盘告警
Y18 7槽位拔盘告警
Y19 8槽位拔盘告警
Y20 9槽位拔盘告警
Y21 10槽位拔盘告警
Y22 11槽位拔盘告警
Y24 12槽位拔盘告警
Y24 13槽位拔盘告警
Y25 14槽位拔盘告警
*/
u8_t mgrAlm[8];
/*版本信息*/
u8_t mgrVer[23];
};
struct extout
{
/* 时钟等级 */
u8_t outSsm[3];
/*
信号类型
0:2.048Mhz
1:2.048Mbit/s
2:无输出
*/
u8_t outSignal[17];
/*
第一个字节表示<mode>,第二个字节表示<out-sta>
<mode> ::= 0|1,普通模式|主备模式
<out-sta> ::= 0|1,主用|备用
*/
u8_t outPR[4];
/*
告警,6个字节
0100Y3Y2Y1Y0 0100Y7Y6Y5Y4 0100Y11Y10Y9Y8
0100Y15Y14Y13Y12 0100Y19Y18Y17Y16 0100Y23Y22Y21Y20
Y0 主钟10M输入告警
Y1 备钟10M输入告警
Y2~Y17 1~16(路/组)输出告警
*/
u8_t outAlm[7];
/*版本信息*/
u8_t outVer[23];
};
struct extsave
{
/*
'E' FLASH已保存配置
*/
u8_t flashMark;
/*
1 主DRV
2 备DRV
*/
u8_t drvPR[EXT_DRV_NUM][EXT_GRP_NUM];
/*
1 主MGR
2 备MGR
*/
u8_t mgrPR[EXT_MGR_NUM];
/* 时钟等级 */
u8_t outSsm[EXT_OUT_NUM][3];
/*
信号类型
0:2.048Mhz
1:2.048Mbit/s
2:无输出
*/
u8_t outSignal[EXT_OUT_NUM][17];
/*
第一个字节表示<mode>,第二个字节表示<out-sta>
<mode> ::= 0|1,普通模式|主备模式
<out-sta> ::= 0|1,主用|备用
*/
u8_t outPR[EXT_OUT_NUM][4];
unsigned char onlineSta;
};
struct extctx
{
//ext save
struct extsave save;
// MCP和DRV的通信告警
// 1 告警
// 0 正常
u8_t extMcpDrvAlm[2];
/* 2张DRV */
struct extdrv drv[EXT_DRV_NUM];
/* 板卡类型,O 无盘,M PWR,f MGR,J OUT16,a OUT32 */
u8_t extBid[EXT_GRP_NUM][15];
/* 6张MGR */
struct extmgr mgr[EXT_MGR_NUM];
/* 30张输出板卡 */
struct extout out[EXT_OUT_NUM];
u8_t old_drv_mgr_sta[32];
u8_t new_drv_mgr_sta[32];
};
#endif//__EXT_CTX__
<file_sep>/MCPU/mcp_main.h
#ifndef _MCP_MAIN_H
#define _MCP_MAIN_H
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
//#include <linux/if.h>
#include <net/route.h>
#include <sys/ioctl.h>
#include <sys/socket.h>
#include <sys/types.h>
#include <sys/time.h>
#include <fcntl.h>
#include <errno.h>
#include "version.h"
#define PORT 5000 //服务器端口
#define BACKLOG 5 //listen队列中等待的连接数
#define MAXRECVDATA 1024
#define MAXDATASIZE 256 //缓冲区大小
#define SENDBUFSIZE 168
//#define _CMD_NODE_MAX 70 //命令条数//modified 2013-4-27
//#define _CMD_NODE_MAX ((int)(sizeof(g_cmd_fun)/sizeof(_CMD_NODE)))
#define PROJ_ID 32
#define PATH_NAME "/var/"
#define MAX_CLIENT 11
//#define _NUM_RPT100S 45 //
#define _NUM_REVT 48 //
#define SAVE_ALM_NUM 50
#define CONFIG_FILE "/usr/config.txt"
#define CR 0x0D //回车
#define LF 0x0A //换行
#define SP 0x20 //空格
#define MCP_SLOT 0x75 //即u
#define GBTP1_SLOT 0x71 //q
#define GBTP2_SLOT 0x72 //r
#define RB1_SLOT 0x6f //o
#define RB2_SLOT 0x70 //p
#ifndef INT_EXTINT0
#define INT_EXTINT0 0x00000000
#endif
#ifndef INT_EXTINT1
#define INT_EXTINT1 0x00000001
#endif
#define FPGA_DEV "/dev/p210fpga"
#define FILE_DEV "/var/p300file"
#define NO_INFO 0
#define DEBUG_NET_INFO 1
#define API_DEF 1
#define NET_INFO "<NET-INFO>"
#define NET_SAVE "<NET-SAVE>"
#define TYPE_MN "MN"
#define TYPE_MJ "MJ"
#define TYPE_CL "CL"
#define TYPE_CR "CR"
#define TYPE_WA "WA"
#define TYPE_MN_A "* "
#define TYPE_MJ_A "**"
#define TYPE_CL_A "A "
#define TYPE_CR_A "*C"
#define TYPE_WA_A "*W"
#define COMMA_1 ","
#define COMMA_3 ",,,"
#define COMMA_7 ",,,,,,,"
#define COMMA_8 ",,,,,,,,"
#define COMMA_9 ",,,,,,,,,"
#define GBTP_G "G,00"
#define GBTP_B "B,00"
#define GBTP_GPS "GPS,00"
#define GBTP_BD "BD,00"
#define GBTP_GLO "GLO,00"
#define GBTP_GAL "GAL,00"
struct mymsgbuf
{
long msgtype;
unsigned char slot;
int len;
unsigned char ctag[7];
unsigned char ctrlstring[SENDBUFSIZE];
};
//网管下发命令结构体
typedef struct
{
unsigned char cmd[16];
int cmd_len;
unsigned char solt;
unsigned char ctag[7];
unsigned char data[MAXDATASIZE];
} _MSG_NODE, *P_MSG_NODE;
typedef struct
{
unsigned char cmd[14];
int cmd_len;
int (*cmd_fun)(_MSG_NODE *, int);
int (*rpt_fun)(int , _MSG_NODE * );
} _CMD_NODE, *P_CMD_NODE;
typedef struct
{
//
//!RPT100S cmd 增加到12
//
unsigned char cmd[12];
int cmd_len;
unsigned char cmd_num[4];
int (*rpt_fun)(char , unsigned char *);
} _RPT100S_NODE;
typedef struct
{
unsigned char cmd_num[4];
int (*rpt_fun)(char , unsigned char *);
} _REVT_NODE;
extern _CMD_NODE g_cmd_fun[];
extern _RPT100S_NODE RPT100S_fun[];
extern _REVT_NODE REVT_fun[];
extern int print_switch;
extern char TOD_STA;
#endif
<file_sep>/MCPU/ext_cmn.c
#include "ext_cmn.h"
//#include "memwatch.h"
int strlen_r( char *str , char end)
{
int len = 0;
while( (*str++) != end )
{
len++;
}
return len;
}
<file_sep>/MCPU/mcp_process.h
#ifndef _MCP_PROCESS_H_
#define _MCP_PROCESS_H_
#include "ext_type.h"
#include "mcp_main.h"
extern int shake_hand_count;
extern int reset_network_card;
int send_tod(unsigned char *buf, unsigned char slot);
int get_tod_sta(unsigned char *buf, unsigned char slot);
extern int strncmp1(unsigned char *p1, unsigned char *p2, unsigned char n);
extern void strncpynew(unsigned char *str1, unsigned char *str2, int n);
extern void strncatnew(unsigned char *str1, unsigned char *str2, int n);
int current_client;
int g_lev;
int init_timer();
void init_pan(char slot);
int judge_length(unsigned char *buf);
void sendto_all_client(unsigned char *buf);
int judge_client_online(int *clt_num);
void judge_data(unsigned char *recvbuf, int len , int client);
int judge_buff(unsigned char *buf);
int IsLeapYear(int year);
void init_var(void);
int Open_FpgaDev(void);
void Close_FpgaDev(void);
int FpgaRead( const long paddr, unsigned short *DstAddr);
int FpgaWrite( const long paddr, unsigned short value);
int process_config();
int process_client(int client, char *recvbuf, int len); //客户请求处理函数
int process_report(unsigned char *recv, int len);
int process_ralm(unsigned char *recv, int len);
int process_revt(unsigned char *recv, int len);
void rpt_alm_to_client(char slot, unsigned char *data);
void send_online_framing(int num, char type);
void get_alm(char slot, unsigned char *data);
int get_time(unsigned char *data);
int get_fpga_ver(unsigned char *data);
int send_pullout_alm(int num, int flag_cl);
void get_time_fromnet();
void get_data(char slot, unsigned char *data);
void rpt_rb(char slot, unsigned char *data);
void rpt_gbtp(char slot, unsigned char *data);
void rpt_out(char slot, unsigned char *data);
void rpt_mcp(unsigned char *data);
void rpt_test(char slot, unsigned char *data);
void rpt_drv(char slot, unsigned char *data);
void send_alm(char slot, int num, char type, int flag_cl);
void save_alm(char slot, unsigned char *data, int flag);
void rpt_alm_framing(char slot, unsigned char *ntfcncde,
unsigned char *almcde, unsigned char *alm);
void get_gbtp_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_gtp_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_btp_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_rb_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_TP16_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_OUT16_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_PTP_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_PGEIN_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_PGE4V_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);//
void get_tod_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_ntp_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_drv_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_mge_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_MCP_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
void get_REF_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num);
int cmd_process(int client, _MSG_NODE *msg);
void respond_success(int client, unsigned char *ctag);
void respond_fail(int client, unsigned char *ctag, int type);
int get_format(char *format, unsigned char *ctag);
int if_a_string_is_a_valid_ipv4_address(const char *str);
void Init_filesystem();
void config_enet();
extern void save_config();
extern int API_Set_McpIp(unsigned char *mcp_local_ip);
extern int API_Set_McpMask(unsigned char *mcp_local_mask);
extern int API_Set_McpGateWay(unsigned char *mcp_local_gateway);
extern int API_Set_McpMacAddr(unsigned char *mcp_local_mac);
extern int API_Get_McpIp(unsigned char *mcp_local_ip);
extern int API_Get_McpMask(unsigned char *mcp_local_mask);
extern int API_Get_McpGateWay(unsigned char *mcp_local_gateway);
extern int API_Get_McpMacAddr(unsigned char *mcp_local_mac);
extern void sendtodown(unsigned char *buf, unsigned char slot);
void rpt_event_framing(unsigned char *event, char slot,
int flag_na, unsigned char *data, int flag_sa, unsigned char *reason);
void send_out_lev(int flag_lev, int num);
int get_freq_lev(int lev);
int get_out_now_lev(unsigned char *parg);
void get_out_now_lev_char(int lev );
void get_out_lev(int num, int flag);
int get_ref_tl(unsigned char c);
int send_ref_lev(int num);
int file_exists(char *filename);
int msgQ_create( const char *pathname, char proj_id );
void sendtodown_cli(unsigned char *buf, unsigned char slot, unsigned char *ctag);
int ext_issue_cmd(u8_t *buf, u16_t len, u8_t slot, u8_t *ctag);
void get_ext1_eqt(char slot, unsigned char *data);
void get_ext2_eqt(char slot, unsigned char *data);
void get_ext3_eqt(char slot, unsigned char *data);
void get_ext_alm(char slot, unsigned char *data);
void get_ext1_alm(char slot, unsigned char *data);
void get_ext2_alm(char slot, unsigned char *data);
void get_ext3_alm(char slot, unsigned char *data);
int ext_alm_cmp(u8_t ext_sid, u8_t *ext_new_data, struct extctx *ctx);
int ext_alm_evt(u8_t eid, u8_t sid, u8_t almid, u8_t *agcc1, u8_t *agcc2);
int ext_bpp_cfg(u8_t ext_sid, u8_t *ext_new_type, struct extctx *ctx);
void ext_bpp_evt(int aid, char bid);
int ext_drv_mgr_evt(struct extctx *ctx);
int ext_bid_cmp(u8_t ext_sid, u8_t *ext_new_data, struct extctx *ctx);
void ext_ssm_cfg(char *pSsm);
void mcpd_reset_network_card(void);
void timer_mcp(void * loop);
int Create_Thread(bool_t * loop);
void get_exta1_eqt(char slot, unsigned char *data);
void get_exta2_eqt(char slot, unsigned char *data);
void get_exta3_eqt(char slot, unsigned char *data);
#endif
<file_sep>/MCPU/mcp_set_struct.h
#ifndef _MCP_SET_STRUCT_H_
#define _MCP_SET_STRUCT_H_
typedef struct
{
unsigned char ip[16];
unsigned char mask[16];
unsigned char gate[16];
unsigned char dns1[16];
unsigned char dns2[16];
unsigned char mac[18];
unsigned char leap_num[3];
unsigned char tid[17];
unsigned char time_source; //MCP盘时间源
unsigned char msmode[5]; //MCP主备用状态
unsigned char tl[3];
unsigned char fb;
/*'0' / '1' 默认为'1'(打开)*/
unsigned char out_ssm_en;
/*默认为0x04*/
unsigned char out_ssm_oth;
} mcp_content;
typedef struct
{
unsigned char mode[4];
unsigned char mask[3];
unsigned char pos[35];
unsigned char bdzb[8];
//unsigned char delay[8];
//unsigned char out_pps_delay[8];
} gbtp_content;
typedef struct
{
unsigned char msmode[5];
unsigned char sys_ref;
unsigned char dely[4][9];
unsigned char priority[9];//the front of reference source priority.
unsigned char mask[9];
unsigned char leapmask[6];
unsigned char tzo[4];
unsigned char leaptag;
unsigned char thresh[2][9];
} rb_content;
typedef struct
{
unsigned char ptp_type;
unsigned char out_lev[3];
unsigned char ptp_linkmode[4];
unsigned char ptp_enp[4];
unsigned char ptp_step[4];
unsigned char ptp_en[4];
unsigned char ptp_delaytype[4];
unsigned char ptp_multicast[4];
unsigned char out_typ[17];
unsigned char out_mod;
unsigned char tp16_en[17];
unsigned char ptp_sync[4][3];
unsigned char ptp_announce[4][3];
unsigned char ptp_delayreq[4][3];
unsigned char ptp_pdelayreq[4][3];
unsigned char ptp_delaycom[4][9];
unsigned char ptp_ip[4][16];
unsigned char ptp_gate[4][16];
unsigned char ptp_mask[4][16];
unsigned char ptp_dns1[4][16];
unsigned char ptp_dns2[4][16];
unsigned char ptp_mac[4][18];
unsigned char ptp_out[4];
unsigned char ref_prio1[9];
unsigned char ref_prio2[9];
unsigned char ref_mod1[4];
unsigned char ref_mod2[4];
unsigned char ref_in_ssm_en[9];
unsigned char ref_2mb_lvlm;
unsigned char rs_en[5];
unsigned char rs_tzo[5];
unsigned char ppx_mod[17];
unsigned char tod_en[17];
unsigned char tod_br;
unsigned char tod_tzo;
unsigned char igb_en[5];
unsigned char igb_rat[5];
unsigned char igb_max[5];
unsigned char ntp_ip[9];
unsigned char ntp_mac[13];
unsigned char ntp_gate[9];
unsigned char ntp_msk[9];
unsigned char ntp_bcast_en;
unsigned char ntp_tpv_en;
unsigned char ntp_md5_en;
unsigned char ntp_ois_en;
unsigned char ntp_interval[5];
unsigned char ntp_key_flag[4];
unsigned char ntp_keyid[4][5];
unsigned char ntp_key[4][17];
/*unsigned char out_type;*/
} out_content;
#define alm_msk_total 50
struct ALM_MSK
{
unsigned char data[24];
unsigned char type; //type 类型,拼写错了
};
typedef struct
{
//'M'表示保存过配置
u8_t flashMask;
//所有要保存设置的信息
mcp_content slot_u;
gbtp_content slot_q, slot_r;
rb_content slot_o, slot_p;
out_content slot[14];
struct ALM_MSK alm_msk[alm_msk_total];
} file_content;
file_content conf_content;
typedef struct
{
unsigned char ip[9];
unsigned char gate[9];
unsigned char mask[9];
unsigned char dns1[9];
unsigned char dns2[9];
unsigned char mac[18];
} STR_STA1;
typedef struct
{
u8_t flashMask;
unsigned char ptp_prio1[14][4][5]; //14个槽位,4个端口,5个字节保存信息。
unsigned char ptp_prio2[14][4][5];
unsigned char LeapMod;
unsigned char ptp_mtc_ip[14][4][9];//保存单播ip
unsigned char ptp_dom[14][4][4]; //20170616新添时钟域号保存
unsigned char ptp_esmcen[14][4]; //ptp esmcen .
unsigned char ptp_sfp[14][4][3];
unsigned char ptp_oplo[14][4][7];
unsigned char ptp_ophi[14][4][7];
unsigned char irigb_tzone[14];
STR_STA1 sta1;
unsigned char mcp_protocol;
unsigned char gbtp_delay[2][8];
unsigned char gbtp_pps_delay[2][16];
unsigned char pps_sel[2];
unsigned char tdev_en[2];
unsigned char ptp_mtc1_ip[14][4][152];
//unsigned char rbb_priority[9]; //#sb the back of reference source priority.
} file_content3; //第一部分配置信息由file_content保存,第二部分为扩展框,此为第三部分。
file_content3 conf_content3;
#define ALM_MASK_LEN 6
#define MAX_PORT_NUM 52
#define MAX_MAC_LEN 12
#define MAX_IP_LEN 8
#define FRAME_FRE_LEN 2
#define DELAY_COM_LEN 7
#define MAX_PRIO_LEN 4
#define MAX_DOM_LEN 3
#define MAX_OPTHR_LEN 6
#define MAX_TIMES_LEN 4
#define MAX_CLKCLS_LEN 3
#define MAX_SYNCSSM_LEN 13
#define PGE4S_ALM5_START 23
#define PGE4S_TALM5_START 22
#endif
<file_sep>/MCPU/version.h
#ifndef _VERSION_H_
#define _VERSION_H_
//主版本号
#define VER_MAJOR "12"
//子版本号
#define VER_MINOR "11"
//修订版本号
#define VER_REVIS "0"
//版本完成日期
#define VER_DATE "190218"
//版本标识
#define VER_STATE 'r' //正式发布版本
//#define VER_STATE 't'
#define MCP_VER "v"VER_MAJOR"."VER_MINOR
#endif<file_sep>/MCPU/ext_alloc.h
#ifndef __EXT_ALLOC__
#define __EXT_ALLOC__
#include "ext_ctx.h"
int initializeContext(struct extctx *ctx);
int ext_read_flash(struct extsave *psave);
int ext_write_flash(struct extsave *psave);
void ext_drv2_mgr6_pr(struct extctx *ctx, u8_t bid13, u8_t bid14);
#endif//__EXT_ALLOC__
<file_sep>/MCPU/ext_alloc.c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include "ext_alloc.h"
#include "mcp_set_struct.h"
//#include "memwatch.h"
/*
1 成功
0 失败
*/
int initializeContext(struct extctx *ctx)
{
int i, ret;
memset(ctx, 0, sizeof(struct extctx));
//drv主备状态初始化
for(i = 0; i < EXT_GRP_NUM; i++)
{
ctx->drv[0].drvPR[i] = 1;
}
for(i = 0; i < EXT_GRP_NUM; i++)
{
ctx->drv[1].drvPR[i] = 2;
}
//mgr主备状态初始化
for(i = 0; i < EXT_MGR_NUM; i += 2)
{
ctx->mgr[i].mgrPR = 1;
ctx->mgr[i + 1].mgrPR = 2;
}
for(i = 0; i < EXT_GRP_NUM; i++)
{
memcpy(ctx->extBid[i], "OOOOOOOOOOOOOO", 14);
}
for(i = 0; i < EXT_DRV_NUM; i++)
{
ctx->drv[i].drvAlm = '@';
}
for(i = 0; i < EXT_MGR_NUM; i++)
{
memcpy(ctx->mgr[i].mgrAlm, "@@@@@@@", 7);
}
for(i = 0; i < EXT_OUT_NUM; i++)
{
memcpy(ctx->out[i].outAlm, "@@@@@@", 6);
}
ret = ext_read_flash(&(ctx->save));
printf("return=%d\n", ret);
printf("p300-1 flashmark=%c\n", ctx->save.flashMark);
if( (1 == ret) && ('F' == ctx->save.flashMark) )
{
printf("%s\n", "read config from flash");
for(i = 0; i < EXT_OUT_NUM; i++)
{
memcpy(ctx->out[i].outSsm, ctx->save.outSsm[i], 2);
memcpy(ctx->out[i].outSignal, ctx->save.outSignal[i], 16);
memcpy(ctx->out[i].outPR, ctx->save.outPR[i], 3);
}
}
else
{
for(i = 0; i < EXT_OUT_NUM; i++)
{
memcpy(ctx->out[i].outSsm, "04", 2);
memcpy(ctx->out[i].outSignal, "1111111111111111", 16);
memcpy(ctx->out[i].outPR, "0,0", 3);
}
memset(&ctx->save, 0, sizeof(struct extsave));
for(i = 0; i < EXT_OUT_NUM; i++)
{
memcpy(ctx->save.outSsm[i], ctx->out[i].outSsm, 2);
memcpy(ctx->save.outSignal[i], ctx->out[i].outSignal, 16);
memcpy(ctx->save.outPR[i], ctx->out[i].outPR, 3);
}
printf("%s\n", "write config to flash");
ctx->save.flashMark = 'F';
if(0 == ext_write_flash(&(ctx->save)))
{
return 0;
}
}
if( (ctx->save.onlineSta & 0xf0) != 0x40)
{
ctx->save.onlineSta = 0x4f;
}
printf("sizeof(struct extsave) = %d\n", (int)sizeof(struct extsave));
printf("sizeof(struct extctx) = %d\n", (int)sizeof(struct extctx));
return 1;
}
/*
1 成功
0 失败
*/
int ext_read_flash(struct extsave *psave)
{
FILE *pf;
u8_t cmd[128];
memset(cmd, 0, 128);
sprintf((char *)cmd, "dd if=/dev/rom1 of=%s bs=65536 count=1 skip=31", EXT_SAVE_PATH);
if(-1 == system(cmd))
{
return 0;
}
sync();
printf("%s SUCCESS\n", cmd);
pf = fopen(EXT_SAVE_PATH, "rb+");
if(NULL == pf)
{
return 0;
}
if(0 != fseek(pf, sizeof(file_content), SEEK_SET))
{
fclose(pf);
return 0;
}
memset(psave, 0, sizeof(struct extsave));
if(1 != fread(psave, sizeof(struct extsave), 1, pf))
{
fclose(pf);
return 0;
}
fclose(pf);
return 1;
}
/*
1 成功
0 失败
*/
int ext_write_flash(struct extsave *psave)
{
FILE *pf;
u8_t cmd[128];
pf = fopen(EXT_SAVE_PATH, "rb+");
if(NULL == pf)
{
return 0;
}
if(0 != fseek(pf, sizeof(file_content), SEEK_SET))
{
fclose(pf);
return 0;
}
if(1 != fwrite(psave, sizeof(struct extsave), 1, pf))
{
fclose(pf);
return 0;
}
fclose(pf);
sync();
memset(cmd, 0, 128);
sprintf((char *)cmd, "fw -ul -f %s -o 0x%x /dev/rom1", EXT_SAVE_PATH, EXT_SAVE_ADDR);
if(-1 == system(cmd))
{
return 0;
}
sync();
printf("%s SUCCESS\n", cmd);
return 1;
}
void ext_drv2_mgr6_pr(struct extctx *ctx, u8_t bid13, u8_t bid14)
{
//drv13
if(EXT_DRV != bid13)
{
ctx->drv[0].drvPR[0] = 4;
ctx->drv[0].drvPR[1] = 4;
ctx->drv[0].drvPR[2] = 4;
}
else
{
//连接状态
if( (ctx->drv[0].drvAlm)&BIT(0) )
{
ctx->drv[0].drvPR[0] = 4;
}
else
{
//通信告警
if(1 == ctx->extMcpDrvAlm[0])
{
ctx->drv[0].drvPR[0] = 3;
}
else
{
ctx->drv[0].drvPR[0] = 1;
}
}
//连接状态
if( (ctx->drv[0].drvAlm)&BIT(1) )
{
ctx->drv[0].drvPR[1] = 4;
}
else
{
//通信告警
if(1 == ctx->extMcpDrvAlm[0])
{
ctx->drv[0].drvPR[1] = 3;
}
else
{
ctx->drv[0].drvPR[1] = 1;
}
}
//连接状态
if( (ctx->drv[0].drvAlm)&BIT(2) )
{
ctx->drv[0].drvPR[2] = 4;
}
else
{
//通信告警
if(1 == ctx->extMcpDrvAlm[0])
{
ctx->drv[0].drvPR[2] = 3;
}
else
{
ctx->drv[0].drvPR[2] = 1;
}
}
}
//drv14
if(EXT_DRV != bid14)
{
ctx->drv[1].drvPR[0] = 4;
ctx->drv[1].drvPR[1] = 4;
ctx->drv[1].drvPR[2] = 4;
}
else
{
//连接状态
if( (ctx->drv[1].drvAlm)&BIT(0) )
{
ctx->drv[1].drvPR[0] = 4;
}
else
{
//通信告警
if(1 == ctx->extMcpDrvAlm[1])
{
ctx->drv[1].drvPR[0] = 3;
}
else
{
if(1 != ctx->drv[0].drvPR[0])
{
ctx->drv[1].drvPR[0] = 1;
}
else
{
ctx->drv[1].drvPR[0] = 2;
}
}
}
//连接状态
if( (ctx->drv[1].drvAlm)&BIT(1) )
{
ctx->drv[1].drvPR[1] = 4;
}
else
{
//通信告警
if(1 == ctx->extMcpDrvAlm[1])
{
ctx->drv[1].drvPR[1] = 3;
}
else
{
if(1 != ctx->drv[0].drvPR[1])
{
ctx->drv[1].drvPR[1] = 1;
}
else
{
ctx->drv[1].drvPR[1] = 2;
}
}
}
//连接状态
if( (ctx->drv[1].drvAlm)&BIT(2) )
{
ctx->drv[1].drvPR[2] = 4;
}
else
{
//通信告警
if(1 == ctx->extMcpDrvAlm[1])
{
ctx->drv[1].drvPR[2] = 3;
}
else
{
if(1 != ctx->drv[0].drvPR[2])
{
ctx->drv[1].drvPR[2] = 1;
}
else
{
ctx->drv[1].drvPR[2] = 2;
}
}
}
}
if((4 == ctx->drv[0].drvPR[0]) && (4 == ctx->drv[1].drvPR[0]))
{
memcpy(ctx->extBid[0], "OOOOOOOOOOOOOO", 14);
}
if((4 == ctx->drv[0].drvPR[1]) && (4 == ctx->drv[1].drvPR[1]))
{
memcpy(ctx->extBid[1], "OOOOOOOOOOOOOO", 14);
}
if((4 == ctx->drv[0].drvPR[2]) && (4 == ctx->drv[1].drvPR[2]))
{
memcpy(ctx->extBid[2], "OOOOOOOOOOOOOO", 14);
}
}
<file_sep>/MCPU/ext_global.c
#include "ext_global.h"
//#include "memwatch.h"
struct extctx gExtCtx;
<file_sep>/MCPU/ext_crpt.c
#include <stdio.h>
#include <string.h>
#include "ext_ctx.h"
#include "ext_crpt.h"
//#include "memwatch.h"
#define MGR_RALM_NUM 4
/*
1 成功
0 失败
*/
u8_t ext_out_ssm_crpt(u8_t save_flag, u8_t ext_sid, u8_t *ext_data, struct extctx *ctx)
{
int _sid;
if((ext_sid >= EXT_1_OUT_LOWER) && (ext_sid <= EXT_1_OUT_UPPER))
{
_sid = ext_sid - EXT_1_OUT_LOWER;
if( EXT_OUT16 == ctx->extBid[0][_sid] ||
EXT_OUT32 == ctx->extBid[0][_sid] ||
EXT_OUT16S == ctx->extBid[0][_sid] ||
EXT_OUT32S == ctx->extBid[0][_sid] )
{
memcpy(ctx->out[ext_sid - EXT_1_OUT_OFFSET].outSsm, ext_data, 2);
if(save_flag)
{
memcpy(ctx->save.outSsm[ext_sid - EXT_1_OUT_OFFSET], ext_data, 2);
}
}
}
else if((ext_sid >= EXT_2_OUT_LOWER) && (ext_sid <= EXT_2_OUT_UPPER))
{
_sid = ext_sid - EXT_2_OUT_LOWER;
if( EXT_OUT16 == ctx->extBid[1][_sid] ||
EXT_OUT32 == ctx->extBid[1][_sid] ||
EXT_OUT16S == ctx->extBid[1][_sid] ||
EXT_OUT32S == ctx->extBid[1][_sid] )
{
memcpy(ctx->out[ext_sid - EXT_2_OUT_OFFSET].outSsm, ext_data, 2);
if(save_flag)
{
memcpy(ctx->save.outSsm[ext_sid - EXT_2_OUT_OFFSET], ext_data, 2);
}
}
}
else if((ext_sid >= EXT_3_OUT_LOWER) && (ext_sid <= EXT_3_OUT_UPPER))
{
if(ext_sid < 58)//except ':;'
{
_sid = ext_sid - EXT_3_OUT_LOWER;
if( EXT_OUT16 == ctx->extBid[2][_sid] ||
EXT_OUT32 == ctx->extBid[2][_sid] ||
EXT_OUT16S == ctx->extBid[2][_sid] ||
EXT_OUT32S == ctx->extBid[2][_sid])
{
memcpy(ctx->out[ext_sid - EXT_3_OUT_OFFSET].outSsm, ext_data, 2);
if(save_flag)
{
memcpy(ctx->save.outSsm[ext_sid - EXT_3_OUT_OFFSET], ext_data, 2);
}
}
}
else
{
_sid = ext_sid - EXT_3_OUT_LOWER - 2;
if( EXT_OUT16 == ctx->extBid[2][_sid] ||
EXT_OUT32 == ctx->extBid[2][_sid] ||
EXT_OUT16S == ctx->extBid[2][_sid] ||
EXT_OUT32S == ctx->extBid[2][_sid] )
{
memcpy(ctx->out[ext_sid - EXT_3_OUT_OFFSET - 2].outSsm, ext_data, 2);
if(save_flag)
{
memcpy(ctx->save.outSsm[ext_sid - EXT_3_OUT_OFFSET - 2], ext_data, 2);
}
}
}
}
else
{
return 0;
}
return 1;
}
/*
1 成功
0 失败
*/
u8_t ext_out_signal_crpt(u8_t save_flag, u8_t ext_sid, u8_t *ext_data, struct extctx *ctx)
{
int _sid;
if((ext_sid >= EXT_1_OUT_LOWER) && (ext_sid <= EXT_1_OUT_UPPER))
{
_sid = ext_sid - EXT_1_OUT_LOWER;
if( EXT_OUT16 == ctx->extBid[0][_sid] ||
EXT_OUT32 == ctx->extBid[0][_sid] ||
EXT_OUT16S == ctx->extBid[0][_sid] ||
EXT_OUT32S == ctx->extBid[0][_sid])
{
memcpy(ctx->out[ext_sid - EXT_1_OUT_OFFSET].outSignal, ext_data, 16);
if(save_flag)
{
memcpy(ctx->save.outSignal[ext_sid - EXT_1_OUT_OFFSET], ext_data, 16);
}
}
}
else if((ext_sid >= EXT_2_OUT_LOWER) && (ext_sid <= EXT_2_OUT_UPPER))
{
_sid = ext_sid - EXT_2_OUT_LOWER;
if( EXT_OUT16 == ctx->extBid[1][_sid] ||
EXT_OUT32 == ctx->extBid[1][_sid] ||
EXT_OUT16S == ctx->extBid[1][_sid] ||
EXT_OUT32S == ctx->extBid[1][_sid] )
{
memcpy(ctx->out[ext_sid - EXT_2_OUT_OFFSET].outSignal, ext_data, 16);
if(save_flag)
{
memcpy(ctx->save.outSignal[ext_sid - EXT_2_OUT_OFFSET], ext_data, 16);
}
}
}
else if((ext_sid >= EXT_3_OUT_LOWER) && (ext_sid <= EXT_3_OUT_UPPER))
{
if(ext_sid < 58)//except ':;'
{
_sid = ext_sid - EXT_3_OUT_LOWER;
if( EXT_OUT16 == ctx->extBid[2][_sid] ||
EXT_OUT32 == ctx->extBid[2][_sid] ||
EXT_OUT16S == ctx->extBid[2][_sid] ||
EXT_OUT32S == ctx->extBid[2][_sid] )
{
memcpy(ctx->out[ext_sid - EXT_3_OUT_OFFSET].outSignal, ext_data, 16);
if(save_flag)
{
memcpy(ctx->save.outSignal[ext_sid - EXT_3_OUT_OFFSET], ext_data, 16);
}
}
}
else
{
_sid = ext_sid - EXT_3_OUT_LOWER - 2;
if( EXT_OUT16 == ctx->extBid[2][_sid] ||
EXT_OUT32 == ctx->extBid[2][_sid] ||
EXT_OUT16S == ctx->extBid[2][_sid] ||
EXT_OUT32S == ctx->extBid[2][_sid] )
{
memcpy(ctx->out[ext_sid - EXT_3_OUT_OFFSET - 2].outSignal, ext_data, 16);
if(save_flag)
{
memcpy(ctx->save.outSignal[ext_sid - EXT_3_OUT_OFFSET - 2], ext_data, 16);
}
}
}
}
else
{
return 0;
}
return 1;
}
/*
1 成功
0 失败
*/
u8_t ext_out_mode_crpt(u8_t save_flag, u8_t ext_sid, u8_t *ext_data, struct extctx *ctx)
{
int _sid;
if((ext_sid >= EXT_1_OUT_LOWER) && (ext_sid <= EXT_1_OUT_UPPER))
{
_sid = ext_sid - EXT_1_OUT_LOWER;
if( EXT_OUT16 == ctx->extBid[0][_sid] ||
EXT_OUT32 == ctx->extBid[0][_sid] ||
EXT_OUT16S == ctx->extBid[0][_sid] ||
EXT_OUT32S == ctx->extBid[0][_sid] )
{
memcpy(ctx->out[ext_sid - EXT_1_OUT_OFFSET].outPR, ext_data, 3);
if(save_flag)
{
memcpy(ctx->save.outPR[ext_sid - EXT_1_OUT_OFFSET], ext_data, 3);
}
}
}
else if((ext_sid >= EXT_2_OUT_LOWER) && (ext_sid <= EXT_2_OUT_UPPER))
{
_sid = ext_sid - EXT_2_OUT_LOWER;
if( EXT_OUT16 == ctx->extBid[1][_sid] ||
EXT_OUT32 == ctx->extBid[1][_sid] ||
EXT_OUT16S == ctx->extBid[1][_sid] ||
EXT_OUT32S == ctx->extBid[1][_sid] )
{
memcpy(ctx->out[ext_sid - EXT_2_OUT_OFFSET].outPR, ext_data, 3);
if(save_flag)
{
memcpy(ctx->save.outPR[ext_sid - EXT_2_OUT_OFFSET], ext_data, 3);
}
}
}
else if((ext_sid >= EXT_3_OUT_LOWER) && (ext_sid <= EXT_3_OUT_UPPER))
{
if(ext_sid < 58)//except ':;'
{
_sid = ext_sid - EXT_3_OUT_LOWER;
if( EXT_OUT16 == ctx->extBid[2][_sid] ||
EXT_OUT32 == ctx->extBid[2][_sid] ||
EXT_OUT16S == ctx->extBid[2][_sid] ||
EXT_OUT32S == ctx->extBid[2][_sid] )
{
memcpy(ctx->out[ext_sid - EXT_3_OUT_OFFSET].outPR, ext_data, 3);
if(save_flag)
{
memcpy(ctx->save.outPR[ext_sid - EXT_3_OUT_OFFSET], ext_data, 3);
}
}
}
else
{
_sid = ext_sid - EXT_3_OUT_LOWER - 2;
if( EXT_OUT16 == ctx->extBid[2][_sid] ||
EXT_OUT32 == ctx->extBid[2][_sid] ||
EXT_OUT16S == ctx->extBid[2][_sid] ||
EXT_OUT32S == ctx->extBid[2][_sid] )
{
memcpy(ctx->out[ext_sid - EXT_3_OUT_OFFSET - 2].outPR, ext_data, 3);
if(save_flag)
{
memcpy(ctx->save.outPR[ext_sid - EXT_3_OUT_OFFSET - 2], ext_data, 3);
}
}
}
}
else
{
return 0;
}
return 1;
}
/*
1 成功
0 失败
*/
u8_t ext_out_prr_crpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx)
{
return 1;
}
/*
1 成功
0 失败
*/
u8_t ext_out_alm_crpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx)
{
if((ext_sid >= EXT_1_OUT_LOWER) && (ext_sid <= EXT_1_OUT_UPPER))
{
memcpy(ctx->out[ext_sid - EXT_1_OUT_OFFSET].outAlm, ext_data, 6);
}
else if((ext_sid >= EXT_2_OUT_LOWER) && (ext_sid <= EXT_2_OUT_UPPER))
{
memcpy(ctx->out[ext_sid - EXT_2_OUT_OFFSET].outAlm, ext_data, 6);
}
else if((ext_sid >= EXT_3_OUT_LOWER) && (ext_sid <= EXT_3_OUT_UPPER))
{
if(ext_sid < 58)//except ':;'
{
memcpy(ctx->out[ext_sid - EXT_3_OUT_OFFSET].outAlm, ext_data, 6);
}
else
{
memcpy(ctx->out[ext_sid - EXT_3_OUT_OFFSET - 2].outAlm, ext_data, 6);
}
}
else if(EXT_1_MGR_PRI == ext_sid)
{
memcpy(ctx->mgr[0].mgrAlm, ext_data, MGR_RALM_NUM);
}
else if(EXT_1_MGR_RSV == ext_sid)
{
memcpy(ctx->mgr[1].mgrAlm, ext_data, MGR_RALM_NUM);
}
else if(EXT_2_MGR_PRI == ext_sid)
{
memcpy(ctx->mgr[2].mgrAlm, ext_data, MGR_RALM_NUM);
}
else if(EXT_2_MGR_RSV == ext_sid)
{
memcpy(ctx->mgr[3].mgrAlm, ext_data, MGR_RALM_NUM);
}
else if(EXT_3_MGR_PRI == ext_sid)
{
memcpy(ctx->mgr[4].mgrAlm, ext_data, MGR_RALM_NUM);
}
else if(EXT_3_MGR_RSV == ext_sid)
{
memcpy(ctx->mgr[5].mgrAlm, ext_data, MGR_RALM_NUM);
}
else
{
return 0;
}
return 1;
}
<file_sep>/MCPU/ext_trpt.h
#ifndef __EXT_TRPT__
#define __EXT_TRPT__
#include "ext_ctx.h"
u8_t ext_out_ssm_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx);
u8_t ext_out_signal_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx);
u8_t ext_out_mode_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx);
u8_t ext_out_bid_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx);
u8_t ext_out_alm_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx);
u8_t ext_out_ver_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx);
u8_t ext_mgr_pr_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx);
#endif//__EXT_TRPT__
<file_sep>/MCPU/mcp_set.c
#include "ext_crpt.h"
#include "ext_global.h"
#include "ext_ctx.h"
#include "mcp_main.h"
#include "mcp_process.h"
#include "ext_alloc.h"
#include "ext_cmn.h"
#include "mcp_def.h"
#include "mcp_set_struct.h"
#include "mcp_save_struct.h"
//#include "memwatch.h"
//#include <sys/ipc.h>
//#include <sys/msg.h>
/* fpga device file descriptor */
static int fpga_fd = -1;
/**
* @brief use linux3.0.x
* @details [long description]
*
*/
typedef struct jiffy_counts_t
{
unsigned long long usr, nic, sys, idle;
unsigned long long iowait, irq, softirq, steal;
unsigned long long total;
unsigned long long busy;
} jiffy_counts_t;
#define CPU_INFO_PATH "/proc/stat"
#define LINE_BUF_NO 500
/**
* @brief cpu_utilization
* @details [long description]
* @return CPU utilization percentage example:return 1 -> 1%
*/
int cpu_utilization(void)
{
static const char fmt[] = "cpu %llu %llu %llu %llu %llu %llu %llu %llu";
char line_buf[LINE_BUF_NO];
jiffy_counts_t jiffy;
jiffy_counts_t jiffyold;
int cpu_util;
int ret;
unsigned long long total_old;
unsigned long long total;
unsigned long long busy_old;
unsigned long long busy;
FILE *fp = fopen(CPU_INFO_PATH, "r");
if (!fgets(line_buf, LINE_BUF_NO, fp))
{
printf("cpu utilization fgets err \n");
}
ret = sscanf(line_buf, fmt,
&jiffyold.usr, &jiffyold.nic, &jiffyold.sys, &jiffyold.idle,
&jiffyold.iowait, &jiffyold.irq, &jiffyold.softirq,
&jiffyold.steal);
busy_old = jiffyold.usr + jiffyold.nic + jiffyold.sys;
total_old = jiffyold.usr + jiffyold.nic + jiffyold.sys + jiffyold.idle;
fclose(fp);
sleep(1);
fp = fopen(CPU_INFO_PATH, "r");
if (!fgets(line_buf, LINE_BUF_NO, fp))
{
printf("cpu utilization fgets err \n");
}
ret = sscanf(line_buf, fmt,
&jiffy.usr, &jiffy.nic, &jiffy.sys, &jiffy.idle,
&jiffy.iowait, &jiffy.irq, &jiffy.softirq,
&jiffy.steal);
busy = jiffy.usr + jiffy.nic + jiffy.sys;
total = jiffy.usr + jiffy.nic + jiffy.sys + jiffy.idle;
cpu_util = (busy - busy_old) * 100.0 / (total - total_old);
#if CPU_UTILIZATION_DEBUG
printf("busy is %llu busy_old is %llu total is %llu total_old is %llu\n", busy, busy_old, total, total_old);
printf("usr is %llu usr_old is %llu \n", jiffyold.usr, jiffy.usr);
printf("cpu is %d \n", cpu_util);
#endif
fclose(fp);
return cpu_util;
}
int Open_FpgaDev(void)
{
if ( ( fpga_fd = open( FPGA_DEV, O_RDWR) ) < 0 )
{
return 0;
}
else
{
return 1;
}
}
/* FPGA 读写函数, 读写FPGA 某一地址的值 */
int FpgaRead( const long paddr, unsigned short *DstAddr)
{
long addr;
int retval;
addr = paddr << 16;
retval = ioctl( fpga_fd, FPGA_READ, &addr );
if ( 0 == retval )
{
*DstAddr = (unsigned short)( addr & 0xFFFF );
return 1;
}
else
{
return 0;
}
}
int FpgaWrite( const long paddr, unsigned short value)
{
long addr;
int retval;
addr = (paddr << 16) + value;
retval = ioctl( fpga_fd, FPGA_WRITE, &addr );
if ( 0 == retval )
{
return 0;
}
else
{
return 1;
}
}
void Close_FpgaDev(void)
{
close(fpga_fd);
}
int cmd_set_tid(_MSG_NODE *msg, int flag)
{
int len = 0;
len = strlen(msg->data);
if(len != 16)
{
return 1;
}
if(strncasecmp(msg->data, "LT", 2) == 0)
{
memset(conf_content.slot_u.tid, '\0', 17);
memcpy(conf_content.slot_u.tid, msg->data, 16);
respond_success(current_client, msg->ctag);
save_config();
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:set_tid:%s\n", NET_INFO, msg->data);
printf("now,tid:%s\n", conf_content.slot_u.tid);
}
#endif
return 0;
}
int cmd_hand_shake(_MSG_NODE *msg, int flag )
{
shake_hand_count++;
//printf("<cmd_hand_shake>\n");
respond_success(current_client, msg->ctag);
timeout_cnt[client_num]++;
if(print_switch == 0)
{
printf("<cmd_hand_shake>client<%d>++\n", client_num);
}
return 0;
}
int cmd_set_main(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
//int len_send;
memset(sendbuf, '\0', SENDBUFSIZE);
if(msg->solt == MCP_SLOT)
{
memset(conf_content.slot_u.msmode, '\0', 5);
memcpy(conf_content.slot_u.msmode, msg->data, 4);
save_config();
}
else if(msg->solt == RB1_SLOT)
{
//if(strcmp(rpt_content.slot_o.rb_msmode,msg->data)!=0)
{
memset(conf_content.slot_o.msmode, '\0', 5);
memcpy(conf_content.slot_o.msmode, msg->data, 4);
/*下发设置*/
/* SET-MAIN:<ctag>::<msmode>;*/
sprintf((char *)sendbuf, "SET-MAIN:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else if(msg->solt == RB2_SLOT)
{
//if(strcmp(rpt_content.slot_p.rb_msmode,msg->data)!=0)
{
memset(conf_content.slot_p.msmode, '\0', 5);
memcpy(conf_content.slot_p.msmode, msg->data, 4);
sprintf((char *)sendbuf, "SET-MAIN:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:set_main:%s\n", NET_INFO, msg->data);
printf("now,msmode:%s\n", conf_content.slot_u.msmode);
}
#endif
return 0;
}
int cmd_set_dat(_MSG_NODE *msg, int flag)
{
/*计算时间差值*/
unsigned char year_s[5], year_rb[5];
unsigned char month_s[3], month_rb[3];
unsigned char day_s[3], day_rb[3];
unsigned char hour_s[3], hour_rb[3];
unsigned char minute_s[3], minute_rb[3];
unsigned char second_s[3], second_rb[3];
int len;
int year, month, day, hour, minute, second;
int year_r, month_r, day_r, hour_r, minute_r, second_r;
if(conf_content.slot_u.time_source == '1')
{
len = strlen(msg->data);
//printf("TEST<1>!\n");
if(len == 21)
/* YYYY-MM-DD HH:mm:SS:f*/
{
memcpy(year_s, msg->data, 4);
memcpy(month_s, &msg->data[5], 2);
memcpy(day_s, &msg->data[8], 2);
memcpy(hour_s, &msg->data[11], 2);
memcpy(minute_s, &msg->data[14], 2);
memcpy(second_s, &msg->data[17], 2);
year = atoi(year_s);
month = atoi(month_s);
day = atoi(day_s);
hour = atoi(hour_s);
minute = atoi(minute_s);
second = atoi(second_s);
if(((hour < 0) || (hour > 23)) || ((minute < 0) || (minute > 60)) || ((second < 0) || (second > 60)))
{
return 1;
}
memcpy(year_rb, mcp_date, 4);
memcpy(month_rb, &mcp_date[5], 2);
memcpy(day_rb, &mcp_date[8], 2);
memcpy(hour_rb, mcp_time, 2);
memcpy(minute_rb, &mcp_time[3], 2);
memcpy(second_rb, &mcp_time[6], 2);
year_r = atoi(year_rb);
month_r = atoi(month_rb);
day_r = atoi(day_rb);
hour_r = atoi(hour_rb);
minute_r = atoi(minute_rb);
second_r = atoi(second_rb);
// printf("TEST<2>!\n");
if((year == year_r) && (month == month_r) && (day == day_r))
{
time_temp = (hour - hour_r) * 3600;
time_temp += (minute - minute_r) * 60;
time_temp += (second - second_r);
printf("time_temp=%d\n", time_temp);
//
}
}
else
{
// printf("TEST<3>!\n");
return 1;
}
}
else
{
// respond_fail(current_client,msg->ctag,0);
return 1;
}
// printf("TEST<4>\n");
respond_success(current_client, msg->ctag);
//save_config();
return 0;
}
int cmd_set_dat_s(_MSG_NODE *msg, int flag)
{
if((msg->data[0] != '1') && (msg->data[0] != '0'))
{
//printf("error dat\n");
return 1;
}
conf_content.slot_u.time_source = msg->data[0];
/*处理*/
respond_success(current_client, msg->ctag);
save_config();
return 0;
}
//设置输出信号SSM 打开,闭塞
int cmd_set_out_ssm_en(_MSG_NODE *msg, int flag)
{
int ret = 1;
printf("SSM enable = %c\n", msg->data[0]);
if(MCP_SLOT == msg->solt)
{
if(('0' == msg->data[0]) || ('1' == msg->data[0]))
{
conf_content.slot_u.out_ssm_en = msg->data[0];
respond_success(current_client, msg->ctag);
save_config();
ret = 0;
}
}
return ret;
}
//
//设置输出信号SSM 门限
//
int cmd_set_out_ssm_oth(_MSG_NODE *msg, int flag)
{
int ret = 1;
unsigned char sendbuf[SENDBUFSIZE];
unsigned char temp = msg->data[0];
printf("SSM threshold = %c\n", msg->data[0]);
if(MCP_SLOT == msg->solt)
{
if((msg->data[0] >= 'a') && (msg->data[0] <= 'f'))
{
switch(msg->data[0])
{
case 'a':
conf_content.slot_u.out_ssm_oth = 0x02;
break;
case 'b':
conf_content.slot_u.out_ssm_oth = 0x04;
break;
case 'c':
conf_content.slot_u.out_ssm_oth = 0x08;
break;
case 'd':
conf_content.slot_u.out_ssm_oth = 0x0b;
break;
case 'e':
conf_content.slot_u.out_ssm_oth = 0x0f;
break;
case 'f':
conf_content.slot_u.out_ssm_oth = 0x00;
break;
}
respond_success(current_client, msg->ctag);
if('S' == slot_type[0])
{
memset(sendbuf, 0, sizeof(sendbuf));
sprintf((char *)sendbuf, "SET-SSM-OTH:%s::%c;", "000000", temp);
sendtodown(sendbuf, 'a');
}
if('S' == slot_type[1])
{
memset(sendbuf, 0, sizeof(sendbuf));
sprintf((char *)sendbuf, "SET-SSM-OTH:%s::%c;", "000000", temp);
sendtodown(sendbuf, 'b');
}
save_config();
ret = 0;
}
}
return ret;
}
int cmd_set_net(_MSG_NODE *msg, int flag)
{
int len, i, j, i1, i2, i3, i4, j1, j2, j3, len_tmp;
int ret;
unsigned char tmp[50];
memset(tmp, '\0', 50);
//
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
j1 = 0;
j2 = 0;
j3 = 0;
if(msg->solt == MCP_SLOT)
{
len = strlen(msg->data);
for(i = 0; i < len; i++)
{
if(msg->data[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
}
}
if(i4 == 0)
{
return 1;
}
//printf("#%s#,%d,%d,%d,%d\n",msg->data,i1,i2,i3,i4);
if(msg->data[0] != ',')
{
memset(tmp, '\0', 50);
len_tmp = i1 - 1;
memcpy(tmp, msg->data, len_tmp);
if(if_a_string_is_a_valid_ipv4_address(tmp) == 0)
{
//if(strcmp(tmp,conf_content.slot_u.ip )!=0)
{
ret = API_Set_McpIp(tmp);
if(ret == 0)
{
memset(conf_content.slot_u.ip, '\0', 16);
memcpy(conf_content.slot_u.ip, tmp, len_tmp);
}
else
{
return 1;
}
}
}
else
{
return 1;
}
}
if(msg->data[i2] != ',')
{
memset(tmp, '\0', 50);
len_tmp = i3 - i2 - 1;
memcpy(tmp, &msg->data[i2], len_tmp);
if(if_a_string_is_a_valid_ipv4_address(tmp) == 0)
{
//if(strcmp(tmp,conf_content.slot_u.mask )!=0)
{
ret = API_Set_McpMask(tmp);
if(ret == 0)
{
memset(conf_content.slot_u.mask, '\0', 16);
memcpy(conf_content.slot_u.mask, tmp, len_tmp);
}
else
{
return 1;
}
}
}
else
{
return 1;
}
}
if(msg->data[i1] != ',')
{
memset(tmp, '\0', 50);
j = 0;
len_tmp = i2 - i1 - 1;
memcpy(tmp, &msg->data[i1], len_tmp);
//printf("gggggggggate=%s\n",tmp);
if(if_a_string_is_a_valid_ipv4_address(tmp) == 0)
{
for(i = 0; i < len_tmp; i++)
{
if(tmp[i] == '.')
{
j++;
if(j == 1)
{
j1 = i;
}
if(j == 2)
{
j2 = i;
}
if(j == 3)
{
j3 = i;
}
}
}
ret = API_Set_McpGateWay(tmp);
if(ret == 0)
{
memset(conf_content.slot_u.gate, '\0', 16);
memcpy(conf_content.slot_u.gate, tmp, len_tmp);
}
else
{
return 1;
}
}
else
{
return 1;
}
}
if(msg->data[i3] != ',')
{
memset(tmp, '\0', 50);
len_tmp = i4 - i3 - 1;
memcpy(tmp, &msg->data[i3], len_tmp);
if(if_a_string_is_a_valid_ipv4_address(tmp) == 0)
{
memset(conf_content.slot_u.dns1, '\0', 16);
memcpy(conf_content.slot_u.dns1, tmp, len_tmp);
}
else
{
return 1;
}
}
if(msg->data[i4] != '\0')
{
memset(tmp, '\0', 50);
len_tmp = len - i4;
memcpy(tmp, &msg->data[i4], len_tmp);
if(if_a_string_is_a_valid_ipv4_address(tmp) == 0)
{
memset(conf_content.slot_u.dns2, '\0', 16);
memcpy(conf_content.slot_u.dns2, tmp, len_tmp);
}
else
{
return 1;
}
}
}
else
{
return 1;
}
respond_success(current_client, msg->ctag);
save_config();
#if DEBUG_NET_INFO
printf("%sip:%s\n", NET_INFO, conf_content.slot_u.ip);
printf("%smask:%s\n", NET_INFO, conf_content.slot_u.mask);
printf("%sgate:%s\n", NET_INFO, conf_content.slot_u.gate);
printf("%sdns1:%s\n", NET_INFO, conf_content.slot_u.dns1);
printf("%sdns2:%s\n", NET_INFO, conf_content.slot_u.dns2);
#endif
return 0;
}
int cmd_set_mac(_MSG_NODE *msg, int flag)
{
int ret;
if(msg->solt == MCP_SLOT)
{
// if(strcmp(msg->data,conf_content.slot_u.mac )!=0)
{
ret = API_Set_McpMacAddr(msg->data);
if(ret == 0)
{
memset(conf_content.slot_u.mac, '\0', 16);
memcpy(conf_content.slot_u.mac, msg->data, 17);
}
else
{
return 1;
}
respond_success(current_client, msg->ctag);
save_config();
}
}
else
{
return 1;
}
return 0;
}
int cmd_set_password(_MSG_NODE *msg, int flag)
{
memset(password, '\0', 9);
memcpy(password, msg->data, 8);
respond_success(current_client, msg->ctag);
save_config();
#if DEBUG_NET_INFO
printf("%s:set_password:%s\n", NET_INFO, msg->data);
printf("now,password:%s\n", password);
#endif
return 0;
}
int cmd_set_mode(_MSG_NODE *msg, int flag)
{
int len;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
len = strlen(msg->data);
if(msg->solt == GBTP1_SLOT)
{
// if(strcmp(rpt_content.slot_q.gb_acmode,msg->data)!=0)
// {
memset(conf_content.slot_q.mode, '\0', 4);
memcpy(conf_content.slot_q.mode, msg->data, len);
/*下发处理*/
/*SET-MODE:<ctag>::<acmode>;*/
sprintf((char *)sendbuf, "SET-MODE:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
// }
}
else if(msg->solt == GBTP2_SLOT)
{
// if(strcmp(rpt_content.slot_r.gb_acmode,msg->data)!=0)
// {
memset(conf_content.slot_r.mode, '\0', 4);
memcpy(conf_content.slot_r.mode, msg->data, len);
sprintf((char *)sendbuf, "SET-MODE:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
// }
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_pos(_MSG_NODE *msg, int flag)
{
int len;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
len = strlen(msg->data);
if(msg->solt == GBTP1_SLOT)
{
//if(strcmp(rpt_content.slot_q.pos,msg->data)!=0)
// {
memset(conf_content.slot_q.pos, '\0', 35);
memcpy(conf_content.slot_q.pos, msg->data, len);
/*下发*//* SET-POS:<ctag>::<postion>;*/
sprintf((char *)sendbuf, "SET-POS:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
//}
}
else if(msg->solt == GBTP2_SLOT)
{
//if(strcmp(conf_content.slot_r.pos,msg->data)!=0)
//{
memset(conf_content.slot_r.pos, '\0', 35);
memcpy(conf_content.slot_r.pos, msg->data, len);
sprintf((char *)sendbuf, "SET-POS:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
//}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_mask_gbtp(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
if((msg->data[0] != '0') && (msg->data[0] != '1'))
{
return 1;
}
if((msg->data[1] != '0') && (msg->data[1] != '1'))
{
return 1;
}
if(msg->solt == GBTP1_SLOT)
{
// if(strcmp(rpt_content.slot_q.gb_mask,msg->data)!=0)
{
memset(conf_content.slot_q.mask, '\0', 3);
memcpy(conf_content.slot_q.mask, msg->data, 2);
/*下发处理*//* SET-MASK:<ctag>::<mask>;*/
sprintf((char *)sendbuf, "SET-MASK:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else if(msg->solt == GBTP2_SLOT)
{
// if(strcmp(rpt_content.slot_r.gb_mask,msg->data)!=0)
{
memset(conf_content.slot_r.mask, '\0', 3);
memcpy(conf_content.slot_r.mask, msg->data, 2);
sprintf((char *)sendbuf, "SET-MASK:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_bdzb(_MSG_NODE *msg, int flag)
{
int len = 0;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
if((strncmp1(msg->data, "BJ54", 4) != 0) && (strncmp1(msg->data, "CGS2000", 7) != 0))
{
return 1;
}
len = strlen(msg->data);
if(msg->solt == GBTP1_SLOT)
{
// if(strcmp(rpt_content.slot_q.gb_mask,msg->data)!=0)
{
memset(conf_content.slot_q.bdzb, '\0', 8);
memcpy(conf_content.slot_q.bdzb, msg->data, len);
sprintf((char *)sendbuf, "SET-BDZB:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else if(msg->solt == GBTP2_SLOT)
{
// if(strcmp(rpt_content.slot_r.gb_mask,msg->data)!=0)
{
memset(conf_content.slot_r.bdzb, '\0', 8);
memcpy(conf_content.slot_r.bdzb, msg->data, len);
sprintf((char *)sendbuf, "SET-BDZB:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_sys_ref(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
//
//!Set sys ref: '0' Auto Select Sys Ref; '1' --- '8' Manual Select Sys Ref; 'F' -----NO Sys Ref;
//
if((*msg->data < 0x30) || ((*msg->data > 0x38) && (*msg->data != 0x46)))
{
return 1;
}
if(msg->solt == RB1_SLOT)
{
//
//!Main Slot Set Sys Ref.
//
conf_content.slot_o.sys_ref = *msg->data;
//
//!Send down Command。
//
sprintf((char *)sendbuf, "SET-SYS-REF:%s::INP-%c;", msg->ctag, *msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
else if(msg->solt == RB2_SLOT)
{
//
//!Slave Slot Set Sys Ref。
//
conf_content.slot_p.sys_ref = *msg->data;
//
//!Send down Command。
//
sprintf((char *)sendbuf, "SET-SYS-REF:%s::INP-%c;", msg->ctag, *msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
else
{
return 1;
}
printf("%s:%s\n", "Set System Ref", sendbuf);
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_dely(_MSG_NODE *msg, int flag)
{
int len, num;
int temp;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
len = strlen(msg->data);
if((msg->data[0] < 0x31) || (msg->data[0] > 0x38))
{
return 1;
}
temp = atoi(&msg->data[2]);
if((temp < 0) || (temp > 99999999))
{
return 1;
}
if(msg->solt == RB1_SLOT)
{
num = (int)(msg->data[0] - 0x31);
if((num >= 0x00) && (num < 4))
{
//if(strcmp(conf_content.slot_o.dely[num], &msg->data[2])!=0)
// {
memset(conf_content.slot_o.dely[num], '\0', 9);
memcpy(conf_content.slot_o.dely[num], &msg->data[2], len - 2);
sprintf((char *)sendbuf, "SET-DELY:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
// }
}
}
else if(msg->solt == RB2_SLOT)
{
num = (int)(msg->data[0] - 0x31);
if((num >= 0x00) && (num < 4))
{
//if(strcmp(rpt_content.slot_p., &msg->data[2])!=0)
// {
memset(conf_content.slot_p.dely[num], '\0', 9);
memcpy(conf_content.slot_p.dely[num], &msg->data[2], len - 2);
sprintf((char *)sendbuf, "SET-DELY:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
// }
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_prio_inp(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int i;
memset(sendbuf, '\0', SENDBUFSIZE);
for(i = 0; i < 8; i++)
{
if((msg->data[i] > '0') && (msg->data[i] < '9'))
{
}
else
{
return 1;
}
}
if(msg->solt == RB1_SLOT)
{
// if(strcmp(rpt_content.slot_o.rb_prio, msg->data)!=0)
{
memset(conf_content.slot_o.priority, '\0', 9);
memcpy(conf_content.slot_o.priority, msg->data, 8);
/*下发*//*SET-PRIO-INP:<ctag>::<priority>;*/
sprintf((char *)sendbuf, "SET-PRIO-INP:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else if(msg->solt == RB2_SLOT)
{
// if(strcmp(rpt_content.slot_p.rb_prio, msg->data)!=0)
{
memset(conf_content.slot_p.priority, '\0', 9);
memcpy(conf_content.slot_p.priority, msg->data, 8);
/*下发*/
sprintf((char *)sendbuf, "SET-PRIO-INP:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_mask_e1(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int i;
memset(sendbuf, '\0', SENDBUFSIZE);
for(i = 0; i < 8; i++)
{
if((msg->data[i] == '0') || (msg->data[i] == '1'))
{}
else
{
return 1;
}
}
if(msg->solt == RB1_SLOT)
{
// if(strcmp(rpt_content.slot_o.rb_mask, msg->data)!=0)
{
memset(conf_content.slot_o.mask, '\0', 9);
memcpy(conf_content.slot_o.mask, msg->data, 8);
/*下发*//*SET-MASK-INP:<ctag>::<mask>;*/
sprintf((char *)sendbuf, "SET-MASK-INP:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else if(msg->solt == RB2_SLOT)
{
// if(strcmp(rpt_content.slot_p.rb_mask, msg->data)!=0)
{
memset(conf_content.slot_p.mask, '\0', 9);
memcpy(conf_content.slot_p.mask, msg->data, 8);
/*下发*/
sprintf((char *)sendbuf, "SET-MASK-INP:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_leap_mask(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int i;
memset(sendbuf, '\0', SENDBUFSIZE);
for(i = 0; i < 5; i++)
{
if((msg->data[i] == '0') || (msg->data[i] == '1'))
{}
else
{
return 1;
}
}
if(msg->solt == RB1_SLOT)
{
// if(strcmp(rpt_content.slot_o.rb_leapmask, msg->data)!=0)
{
memset(conf_content.slot_o.leapmask, '\0', 6);
memcpy(conf_content.slot_o.leapmask, msg->data, 5);
/*下发*//*SET-LMAK-INP:<ctag>::<leapmask>;*/
sprintf((char *)sendbuf, "SET-LMAK-INP:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else if(msg->solt == RB2_SLOT)
{
// if(strcmp(rpt_content.slot_p.rb_leapmask, msg->data)!=0)
{
memset(conf_content.slot_p.leapmask, '\0', 6);
memcpy(conf_content.slot_p.leapmask, msg->data, 5);
/*下发*/
sprintf((char *)sendbuf, "SET-LMAK-INP:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_tzo(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
if((msg->data[0] != '0') && (msg->data[0] != '1'))
{
return 1;
}
if((msg->data[2] < 0x40) || (msg->data[2] > 0x4c))
{
return 1;
}
if(msg->solt == RB1_SLOT)
{
// if(strcmp(rpt_content.slot_o.rf_tzo,msg->data)!=0)
{
memset(conf_content.slot_o.tzo, '\0', 4);
memcpy(conf_content.slot_o.tzo, msg->data, 3);
sprintf((char *)sendbuf, "SET-TZO:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else if(msg->solt == RB2_SLOT)
{
// if(strcmp(rpt_content.slot_p.rf_tzo,msg->data)!=0)
{
memset(conf_content.slot_p.tzo, '\0', 4);
memcpy(conf_content.slot_p.tzo, msg->data, 3);
sprintf((char *)sendbuf, "SET-TZO:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_ptp_net(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num;
int port;
int i, j, i1, i2, i3, i4, i5, i6;
int len = strlen(msg->data);
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
i5 = 0;
i6 = 0;
memset(sendbuf, '\0', SENDBUFSIZE);
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
if((msg->data[2] > '0') && (msg->data[2] < '5'))
{
// memset(conf_content.slot[num].tp16_en,'\0',17);
num = (int)(msg->solt - 0x61);
port = (int)(msg->data[2] - 0x31);
// if(strcmp(conf_content.slot[num].ptp_net[port],msg->data)!=0)
// {
//memset(conf_content.slot[num].ptp_net[port],'\0',99);
//memcpy(conf_content.slot[num].ptp_net[port],msg->data,len);
/*SET-PTP-NET:<type>,<response message>;*/
for(i = 0; i < len; i++)
{
if(msg->data[i] == ',')
{
j++;
if(j == 2)
{
i1 = i + 1;
}
if(j == 3)
{
i2 = i + 1;
}
if(j == 4)
{
i3 = i + 1;
}
if(j == 5)
{
i4 = i + 1;
}
if(j == 6)
{
i5 = i + 1;
}
if(j == 7)
{
i6 = i + 1;
}
}
}
if(i6 == 0)
{
return 1;
}
conf_content.slot[num].ptp_type = msg->data[0];
if(msg->data[i1] != ',')
{
memcpy(conf_content.slot[num].ptp_ip[port], &msg->data[i1], i2 - i1 - 1);
}
if(msg->data[i2] != ',')
{
memcpy(conf_content.slot[num].ptp_mac[port], &msg->data[i2], i3 - i2 - 1);
}
if(msg->data[i3] != ',')
{
memcpy(conf_content.slot[num].ptp_gate[port], &msg->data[i3], i4 - i3 - 1);
}
if(msg->data[i4] != ',')
{
memcpy(conf_content.slot[num].ptp_mask[port], &msg->data[i4], i5 - i4 - 1);
}
if(msg->data[i5] != ',')
{
memcpy(conf_content.slot[num].ptp_dns1[port], &msg->data[i5], i6 - i5 - 1);
}
if(msg->data[i6] != '\0')
{
memcpy(conf_content.slot[num].ptp_dns2[port], &msg->data[i6], len - i6);
}
sprintf((char *)sendbuf, "SET-PTP-NET:%s;", msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
// }
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_ptp_mod(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num;
int port;
int i, j, i1, i2, i3, i4, i5, i6, i7, i8, i9, i10, i11, i12, i13;
int len = strlen(msg->data);
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
i5 = 0;
i6 = 0;
i7 = 0;
i8 = 0;
i9 = 0;
i10 = 0;
i11 = 0;
i12 = 0;
i13 = 0;
memset(sendbuf, '\0', SENDBUFSIZE);
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
if((msg->data[2] > '0') && (msg->data[2] < '5'))
{
//memset(conf_content.slot[num].tp16_en,'\0',17);
num = (int)(msg->solt - 0x61);
port = (int)(msg->data[2] - 0x31);
//if(strcmp(conf_content.slot[num].ptp_mod[port],msg->data)!=0)
// {
// memset(conf_content.slot[num].ptp_mod[port],'\0',34);
// memcpy(conf_content.slot[num].ptp_mod[port],msg->data,len);
/*SET-PTP-MOD:<type>,<response message>;*/
for(i = 0; i < len; i++)
{
if(msg->data[i] == ',')
{
j++;
if(j == 2)
{
i1 = i + 1;
}
if(j == 3)
{
i2 = i + 1;
}
if(j == 4)
{
i3 = i + 1;
}
if(j == 5)
{
i4 = i + 1;
}
if(j == 6)
{
i5 = i + 1;
}
if(j == 7)
{
i6 = i + 1;
}
if(j == 8)
{
i7 = i + 1;
}
if(j == 9)
{
i8 = i + 1;
}
if(j == 10)
{
i9 = i + 1;
}
if(j == 11)
{
i10 = i + 1;
}
if(j == 12)
{
i11 = i + 1;
}
if(j == 13)
{
i12 = i + 1;
}
if(j == 14)
{
i13 = i + 1; //2014.12.2
}
}
}
if(i12 == 0)
{
return 1;
}
conf_content.slot[num].ptp_type = msg->data[0];
if((msg->data[i1] == '0') || (msg->data[i1] == '1'))
{
conf_content.slot[num].ptp_en[port] = msg->data[i1];
}
if((msg->data[i2] == '0') || (msg->data[i2] == '1'))
{
conf_content.slot[num].ptp_delaytype[port] = msg->data[i2];
}
if((msg->data[i3] == '0') || (msg->data[i3] == '1'))
{
//printf("ptp set %c\n",msg->data[i3]);
conf_content.slot[num].ptp_multicast[port] = msg->data[i3];
}
if((msg->data[i4] == '0') || (msg->data[i4] == '1'))
{
conf_content.slot[num].ptp_enp[port] = msg->data[i4];
}
if((msg->data[i5] == '0') || (msg->data[i5] == '1'))
{
conf_content.slot[num].ptp_step[port] = msg->data[i5];
}
if(msg->data[i6] != ',')
{
memcpy(conf_content.slot[num].ptp_sync[port], &msg->data[i6], i7 - i6 - 1);
}
if(msg->data[i7] != ',')
{
memcpy(conf_content.slot[num].ptp_announce[port], &msg->data[i7], i8 - i7 - 1);
}
if(msg->data[i8] != ',')
{
memcpy(conf_content.slot[num].ptp_delayreq[port], &msg->data[i8], i9 - i8 - 1);
}
if(msg->data[i9] != ',')
{
memcpy(conf_content.slot[num].ptp_pdelayreq[port], &msg->data[i9], i10 - i9 - 1);
}
if(msg->data[i10] != ',')
{
memset(conf_content.slot[num].ptp_delaycom[port],'\0',9);//ADD PTP4
memcpy(conf_content.slot[num].ptp_delaycom[port], &msg->data[i10], i11 - i10 - 1);
}
if((msg->data[i11] == '0') || (msg->data[i11] == '1'))
{
conf_content.slot[num].ptp_linkmode[port] = msg->data[i11];
}
if((msg->data[i12] == '0') || (msg->data[i12] == '1'))
{
conf_content.slot[num].ptp_out[port] = msg->data[i12];
}
//2014.12.2
if(i13 != 0 && msg->data[i13] != '\0')
{
if(msg->data[i13] == 'A')
{
memset(conf_content3.ptp_prio1[num][port], 0, 5);
memcpy(conf_content3.ptp_prio1[num][port], (msg->data) + i13, len - i13);
//prio = 0;
}
else if(msg->data[i13] == 'B')
{
memset(conf_content3.ptp_prio2[num][port], 0, 5);
memcpy(conf_content3.ptp_prio2[num][port], (msg->data) + i13, len - i13);
}
else
{
return 1;
}
}
//printf("\n\nLUOHAO~~~~~~~~~2,conf_content.slot[%d].ptp_multicast[%d] = %d\n\n",num,port,conf_content.slot[num].ptp_multicast[port]);
sprintf((char *)sendbuf, "SET-PTP-MOD:%s;", msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
// }
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_ptp_mtc(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num,i;
int port;
int ip_len;
int max_ip_len = sizeof( conf_content3.ptp_mtc1_ip[0][0]);
ip_len = strlen(msg->data)-4;
//
//!if no ip set,return;one ip address 8 byte;
//
if(ip_len < 8 || ip_len > max_ip_len)
return 1;
//
//!check ip array is valid.
//
for(i = 9; i < ip_len; )
{
if(msg->data[i+3] != '.')
return 1;
else
i += 9;
}
memset(sendbuf, '\0', SENDBUFSIZE);
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
if((msg->data[2] > '0') && (msg->data[2] < '5'))
{
num = (int)(msg->solt - 0x61);
port = (int)(msg->data[2] - 0x31);
if(msg->data[4] != '\0')
{
memset(conf_content3.ptp_mtc1_ip[num][port], '\0', max_ip_len);
memcpy(conf_content3.ptp_mtc1_ip[num][port], &msg->data[4], ip_len);
sprintf((char *)sendbuf, "SET-PTP-MTC:%s;", msg->data);
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
return 0;
}
return 1;
}
else
{
return 1;
}
}
int cmd_set_ptp_dom(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num;
int port;
int i;
memset(sendbuf, '\0', SENDBUFSIZE);
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
if((msg->data[2] > '0') && (msg->data[2] < '5'))
{
num = (int)(msg->solt - 0x61);
port = (int)(msg->data[2] - 0x31);
if(msg->data[4] != ';')
{
//printf("set ptp domA:%s \n",msg->data);
for(i = 4; msg->data[i] != '\0' ; i++ )
{
if(i >= 7)
return 1;
}
//printf("set ptp domB:%s \n",msg->data);
memset(conf_content3.ptp_dom[num][port], 0, 4);
memcpy(conf_content3.ptp_dom[num][port], &msg->data[4], i - 4);
sprintf((char *)sendbuf, "SET-PTP-DOM:%s;", msg->data);
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
return 0;
}
return 1;
}
else
{
return 1;
}
}
/*
int cmd_set_ptp_out(_MSG_NODE * msg,int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num;
int port;
memset(sendbuf,'\0',SENDBUFSIZE);
if(((msg->solt)>'`')&&((msg->solt)<'o'))
{
if((msg->data[2]>'0')&&(msg->data[2]<'5'))
{
num=(int)(msg->solt-0x61);
port=(int)(msg->data[2]-0x31);
if((msg->data[4]=='1' )||(msg->data[4]=='0'))
{
conf_content.slot[num].ptp_out[port]=msg->data[4];
}
else
return 1;
sprintf((char *)sendbuf,"SET-PTP-OUT:%s;",msg->data);
if(flag)
{
sendtodown_cli(sendbuf,msg->solt,msg->ctag);
save_config();
}
else
sendtodown(sendbuf, msg->solt);
}
else
return 1;
}
else
return 1;
return 0;
}
*/
int cmd_set_out_lev(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
unsigned char tmp_ctag[7];
int num;
memset(sendbuf, '\0', SENDBUFSIZE);
memset(tmp_ctag, 0, 7);
memcpy(tmp_ctag, "000000", 6);
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
num = (int)(msg->solt - 0x61);
memset(conf_content.slot[num].out_lev, '\0', 3);
memcpy(conf_content.slot[num].out_lev, msg->data, 2);
//SET-OUT-LEV:<ctag>::<level>;
sprintf((char *)sendbuf, "SET-OUT-LEV:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
//ext
else if((msg->solt >= EXT_1_OUT_LOWER && msg->solt <= EXT_1_OUT_UPPER) ||
(msg->solt >= EXT_2_OUT_LOWER && msg->solt <= EXT_2_OUT_UPPER) ||
(msg->solt >= EXT_3_OUT_LOWER && msg->solt <= EXT_3_OUT_UPPER && msg->solt != ':' && msg->solt != ';') )
{
if(msg->solt >= EXT_1_OUT_LOWER && msg->solt <= EXT_1_OUT_UPPER)
{
memcpy(gExtCtx.out[msg->solt - EXT_1_OUT_OFFSET].outSsm, msg->data, 2);
memcpy(gExtCtx.save.outSsm[msg->solt - EXT_1_OUT_OFFSET], msg->data, 2);
}
else if(msg->solt >= EXT_2_OUT_LOWER && msg->solt <= EXT_2_OUT_UPPER)
{
memcpy(gExtCtx.out[msg->solt - EXT_2_OUT_OFFSET].outSsm, msg->data, 2);
memcpy(gExtCtx.save.outSsm[msg->solt - EXT_2_OUT_OFFSET], msg->data, 2);
}
else
{
if(msg->solt < ':')
{
memcpy(gExtCtx.out[msg->solt - EXT_3_OUT_OFFSET].outSsm, msg->data, 2);
memcpy(gExtCtx.save.outSsm[msg->solt - EXT_3_OUT_OFFSET], msg->data, 2);
}
else
{
memcpy(gExtCtx.out[msg->solt - EXT_3_OUT_OFFSET - 2].outSsm, msg->data, 2);
memcpy(gExtCtx.save.outSsm[msg->solt - EXT_3_OUT_OFFSET - 2], msg->data, 2);
}
}
if(flag)
{
sprintf((char *)sendbuf, "SET-OUT-LEV:%c:%s::%s;", msg->solt, msg->ctag, msg->data);
if(0 == ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, msg->solt, msg->ctag))
{
return 1;
}
}
else
{
sprintf((char *)sendbuf, "SET-OUT-LEV:%c:%s::%s;", msg->solt, tmp_ctag, msg->data);
if(0 == ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, msg->solt, tmp_ctag))
{
return 1;
}
}
if(0 == ext_write_flash(&(gExtCtx.save)))
{
return 1;
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_out_ppr(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num;
unsigned char tmp_ctag[7];
memset(sendbuf, '\0', SENDBUFSIZE);
memset(tmp_ctag, 0, 7);
memcpy(tmp_ctag, "000000", 6);
if( ((msg->solt) > '`') && ((msg->solt) < 'o') )
{
num = (int)(msg->solt - 0x61);
sprintf((char *)sendbuf, "SET-OUT-PRR:%s::;", msg->ctag);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
//ext
else if((msg->solt >= EXT_1_OUT_LOWER && msg->solt <= EXT_1_OUT_UPPER) ||
(msg->solt >= EXT_2_OUT_LOWER && msg->solt <= EXT_2_OUT_UPPER) ||
(msg->solt >= EXT_3_OUT_LOWER && msg->solt <= EXT_3_OUT_UPPER && msg->solt != ':' && msg->solt != ';') )
{
if(flag)
{
sprintf((char *)sendbuf, "SET-OUT-PRR:%c:%s::;", msg->solt, msg->ctag);
if(0 == ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, msg->solt, msg->ctag))
{
return 1;
}
}
else
{
sprintf((char *)sendbuf, "SET-OUT-PRR:%c:%s::;", msg->solt, tmp_ctag);
if(0 == ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, msg->solt, tmp_ctag))
{
return 1;
}
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_out_typ(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num, i;
unsigned char tmp_ctag[7];
memset(tmp_ctag, 0, 7);
memcpy(tmp_ctag, "000000", 6);
memset(sendbuf, '\0', SENDBUFSIZE);
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
num = (int)(msg->solt - 0x61);
for(i = 0; i < 16; i++)
{
if((msg->data[i] < '0') || (msg->data[i] > '2'))
{
return 1;
}
}
memset(conf_content.slot[num].out_typ, '\0', 17);
memcpy(conf_content.slot[num].out_typ, msg->data, 16);
/*SET-OUT-TYP:<ctag>::<level>;*/
sprintf((char *)sendbuf, "SET-OUT-TYP:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
else if((msg->solt >= EXT_1_OUT_LOWER && msg->solt <= EXT_1_OUT_UPPER) ||
(msg->solt >= EXT_2_OUT_LOWER && msg->solt <= EXT_2_OUT_UPPER) ||
(msg->solt >= EXT_3_OUT_LOWER && msg->solt <= EXT_3_OUT_UPPER && msg->solt != ':' && msg->solt != ';') )
{
for(i = 0; i < 16; i++)
{
if( (msg->data[i] < '0') || (msg->data[i] > '2') )
{
return 1;
}
}
if(0 == ext_out_signal_crpt(1, msg->solt, msg->data, &gExtCtx))
{
return 1;
}
if(flag)
{
sprintf((char *)sendbuf, "SET-OUT-TYP:%c:%s::%s;", msg->solt, msg->ctag, msg->data);
if(0 == ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, msg->solt, msg->ctag))
{
return 1;
}
}
else
{
sprintf((char *)sendbuf, "SET-OUT-TYP:%c:%s::%s;", msg->solt, tmp_ctag, msg->data);
if(0 == ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, msg->solt, tmp_ctag))
{
return 1;
}
}
if(0 == ext_write_flash(&(gExtCtx.save)))
{
return 1;
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_out_mod(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num;
unsigned char tmp_ctag[7];
memset(sendbuf, '\0', SENDBUFSIZE);
memset(tmp_ctag, 0, 7);
memcpy(tmp_ctag, "000000", 6);
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
if((msg->data[0] == '1') || (msg->data[0] == '0'))
{
num = (msg->solt) - 'a';
conf_content.slot[num].out_mod = msg->data[0];
sprintf((char *)sendbuf, "SET-OUT-MOD:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
else
{
return 1;
}
}
//ext
else if((msg->solt >= EXT_1_OUT_LOWER && msg->solt <= EXT_1_OUT_UPPER) ||
(msg->solt >= EXT_2_OUT_LOWER && msg->solt <= EXT_2_OUT_UPPER) ||
(msg->solt >= EXT_3_OUT_LOWER && msg->solt <= EXT_3_OUT_UPPER && msg->solt != ':' && msg->solt != ';') )
{
if(msg->solt >= EXT_1_OUT_LOWER && msg->solt <= EXT_1_OUT_UPPER)
{
gExtCtx.out[msg->solt - EXT_1_OUT_OFFSET].outPR[0] = msg->data[0];
gExtCtx.save.outPR[msg->solt - EXT_1_OUT_OFFSET][0] = msg->data[0];
}
else if(msg->solt >= EXT_2_OUT_LOWER && msg->solt <= EXT_2_OUT_UPPER)
{
gExtCtx.out[msg->solt - EXT_2_OUT_OFFSET].outPR[0] = msg->data[0];
gExtCtx.save.outPR[msg->solt - EXT_2_OUT_OFFSET][0] = msg->data[0];
}
else
{
if(msg->solt < ':')//except : ;
{
gExtCtx.out[msg->solt - EXT_3_OUT_OFFSET].outPR[0] = msg->data[0];
gExtCtx.save.outPR[msg->solt - EXT_3_OUT_OFFSET][0] = msg->data[0];
}
else
{
gExtCtx.out[msg->solt - EXT_3_OUT_OFFSET - 2].outPR[0] = msg->data[0];
gExtCtx.save.outPR[msg->solt - EXT_3_OUT_OFFSET - 2][0] = msg->data[0];
}
}
if(flag)
{
sprintf((char *)sendbuf, "SET-OUT-MOD:%c:%s::%s;", msg->solt, msg->ctag, msg->data);
if(0 == ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, msg->solt, msg->ctag))
{
return 1;
}
}
else
{
sprintf((char *)sendbuf, "SET-OUT-MOD:%c:%s::%s;", msg->solt, tmp_ctag, msg->data);
if(0 == ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, msg->solt, tmp_ctag))
{
return 1;
}
}
if(0 == ext_write_flash(&(gExtCtx.save)))
{
return 1;
}
}
else
{
return 1;
}
return 0;
}
int cmd_set_reset(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
if((msg->solt == ':') || (msg->solt == 'u'))
{
/****reboot*/
memcpy(sendbuf, "RESET", 5);
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
respond_success(current_client, msg->ctag);
}
else if((msg->solt > '`') && (msg->solt < 's'))
{
sprintf((char *)sendbuf, "SET-RESET:%s::;", msg->ctag);
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
}
else if(msg->solt == 'v')
{}
else if((msg->solt == 's') || ((msg->solt == 't')))
{}
else
{
return 1;
}
/*SET-RESET:<ctag>::;*/
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_leap_tag(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
if(msg->solt == RB1_SLOT)
{
// if(rpt_content.slot_o.rb_leaptag!=*msg->data)
{
conf_content.slot_o.leaptag = *msg->data;
/*SET-LEAP:<ctag>::<leaptag>;*/
sprintf((char *)sendbuf, "SET-LEAP:%s::%c;", msg->ctag, *msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else if(msg->solt == RB2_SLOT)
{
// if(rpt_content.slot_p.rb_leaptag!=*msg->data)
{
conf_content.slot_p.leaptag = *msg->data;
sprintf((char *)sendbuf, "SET-LEAP:%s::%c;", msg->ctag, *msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_tp16_en(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num = 0, i;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
// memset(tmp,'\0',sizeof(unsigned char) * 17);
for(i = 0; i < 16; i++)
{
if((msg->data[i] != '0') && (msg->data[i] != '1'))
{
return 1;
}
}
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
//memset(&conf_content.slot[num],'\0',sizeof(out_content));
num = (int)(msg->solt - 0x61);
// if(strcmp(rpt_content.slot[num].tp16_en, msg->data)!=0)
// {
//memset(conf_content.slot[num].tp16_en, '\0', 17);
// for(i=0;i<16;i++)
// tmp[i]=msg->data[15-i];
memcpy(conf_content.slot[num].tp16_en, msg->data, 16);
/*SET-TP16-EN::<ctag>::<en>;*/
sprintf((char *)sendbuf, "SET-TP16-EN:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
// }
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
// free(tmp);
return 0;
}
int cmd_set_leap_num(_MSG_NODE *msg, int flag)
{
int num, i;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
num = atoi(msg->data);
if(strncmp1(msg->data, "00", 2) == 0)
{}
else if((num < 1) || (num > 99))
{
return 1;
}
if((msg->solt == ':') || (msg->solt == 'u'))
{
memset(conf_content.slot_u.leap_num, '\0', 3);
memcpy(conf_content.slot_u.leap_num, msg->data, 2);
sprintf((char *)sendbuf, "SET-LEAP-NUM:%s::%s;", msg->ctag, msg->data);
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
for(i = 0; i < 14; i++)
{
if(slot_type[i] == 'I')
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-TP16-LEAP:%s::%s;", msg->ctag, msg->data);
sendtodown(sendbuf, i + 0x61);
}
else if((slot_type[i] > '@') && (slot_type[i] < 'I'))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PTP-LEAP:%s::%s;", msg->ctag, msg->data);
sendtodown(sendbuf, i + 0x61);
}
else if(slot_type[i] == 'j')
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PTP-LEAP:%s::%s;", msg->ctag, msg->data);
sendtodown(sendbuf, i + 0x61);
}
else if(slot_type[i] == 'T' || slot_type[i] == 'X')
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-RS-TZO:%s::,%s;", msg->ctag, msg->data);
sendtodown(sendbuf, i + 0x61);
}
else if(slot_type[i] == 'V')
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-TOD-PRO:%s::,,%s;", msg->ctag, msg->data);
sendtodown(sendbuf, i + 0x61);
}
else if(slot_type[i] == 'W')
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-IGB-LEAP:%s::%s;", msg->ctag, msg->data);
sendtodown(sendbuf, i + 0x61);
}
else if(slot_type[i] == 'Z')
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-NTP-LEAP:%s::%s;", msg->ctag, msg->data);
sendtodown(sendbuf, i + 0x61);
}
}
//if the online pan type at the 15 slot, it need to send the leap value to the 15 slot.the 16 slot as the up.
//
if((slot_type[14] == 'R') | (slot_type[14] == 'K'))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-RB-LEAP:%s::%s;", msg->ctag, msg->data);
sendtodown(sendbuf, 14 + 0x61);
}
if((slot_type[15] == 'R') | (slot_type[15] == 'K'))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-RB-LEAP:%s::%s;", msg->ctag, msg->data);
sendtodown(sendbuf, 15 + 0x61);
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, conf_content.slot_u.leap_num);
}
#endif
return 0;
}
int cmd_rtrv_alrms(int client, _MSG_NODE *msg)
{
int send_ok, num;
unsigned char sendbuf[1024];
memset(sendbuf, '\0', sizeof(unsigned char) * 1024);
num = (int)(msg->solt - 'a');
get_format(sendbuf, msg->ctag);
get_alm(msg->solt, sendbuf);
//printf("<%s>:%s\n",__FUNCTION__,sendbuf);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(send_ok == -1 && errno == EAGAIN)
{
printf("timeout_<3>!\n");
}
return 0;
}
/*
0 成功
1 失败
*/
int cmd_ext_alm(int client, _MSG_NODE *msg)
{
int ret;
u8_t sendbuf[1024];
memset(sendbuf, 0, 1024);
get_format(sendbuf, msg->ctag);
get_ext_alm(msg->solt, sendbuf);
ret = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(ret == -1 && errno != EAGAIN)
{
return 1;
}
return 0;
}
/*
0 成功
1 失败
*/
int cmd_ext1_alm(int client, _MSG_NODE *msg)
{
int ret;
u8_t sendbuf[1024];
memset(sendbuf, 0, 1024);
get_format(sendbuf, msg->ctag);
get_ext1_alm(msg->solt, sendbuf);
ret = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(ret == -1 && errno != EAGAIN)
{
return 1;
}
return 0;
}
/*
0 成功
1 失败
*/
int cmd_ext2_alm(int client, _MSG_NODE *msg)
{
int ret;
u8_t sendbuf[1024];
memset(sendbuf, 0, 1024);
get_format(sendbuf, msg->ctag);
get_ext2_alm(msg->solt, sendbuf);
ret = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(ret == -1 && errno != EAGAIN)
{
return 1;
}
return 0;
}
/*
0 成功
1 失败
*/
int cmd_ext3_alm(int client, _MSG_NODE *msg)
{
int ret;
u8_t sendbuf[1024];
memset(sendbuf, 0, 1024);
get_format(sendbuf, msg->ctag);
get_ext3_alm(msg->solt, sendbuf);
ret = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(ret == -1 && errno != EAGAIN)
{
return 1;
}
return 0;
}
/*
0 成功
1 失败
*/
int cmd_ext1_prs(int client, _MSG_NODE *msg)
{
u8_t sendbuf[1024];
int i, ret;
memset(sendbuf, 0, 1024);
get_format(sendbuf, msg->ctag);
sprintf((char *)sendbuf, "%s%c%c%c\"", sendbuf, SP, SP, SP);
for(i = 0; i < 10; i++)
{
if( (EXT_OUT16 == gExtCtx.extBid[0][i]) ||
(EXT_OUT32 == gExtCtx.extBid[0][i]) ||
(EXT_OUT16S == gExtCtx.extBid[0][i]) ||
(EXT_OUT32S == gExtCtx.extBid[0][i]) )
{
sprintf((char *)sendbuf, "%s%c,%s",
sendbuf,
gExtCtx.extBid[0][i],
((0 == strlen(gExtCtx.out[i].outPR)) ? (char *)"," : (char *)(gExtCtx.out[i].outPR)));
}
else
{
sprintf((char *)sendbuf, "%s%c,%s",
sendbuf,
gExtCtx.extBid[0][i],
",");
}
if(i < 9)
{
strcat(sendbuf, ":");
}
}
strcat(sendbuf, "\"\r\n;");
ret = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(ret == -1 && errno != EAGAIN)
{
return 1;
}
return 0;
}
/*
0 成功
1 失败
*/
int cmd_ext2_prs(int client, _MSG_NODE *msg)
{
u8_t sendbuf[1024];
int i, ret;
memset(sendbuf, 0, 1024);
get_format(sendbuf, msg->ctag);
sprintf((char *)sendbuf, "%s%c%c%c\"", sendbuf, SP, SP, SP);
for(i = 0; i < 10; i++)
{
if( (EXT_OUT16 == gExtCtx.extBid[1][i]) ||
(EXT_OUT32 == gExtCtx.extBid[1][i]) ||
(EXT_OUT16S == gExtCtx.extBid[1][i]) ||
(EXT_OUT32S == gExtCtx.extBid[1][i]) )
{
sprintf((char *)sendbuf, "%s%c,%s",
sendbuf,
gExtCtx.extBid[1][i],
((0 == strlen(gExtCtx.out[10 + i].outPR)) ? (char *)"," : (char *)(gExtCtx.out[10 + i].outPR)));
}
else
{
sprintf((char *)sendbuf, "%s%c,%s",
sendbuf,
gExtCtx.extBid[1][i],
",");
}
if(i < 9)
{
strcat(sendbuf, ":");
}
}
strcat(sendbuf, "\"\r\n;");
ret = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(ret == -1 && errno != EAGAIN)
{
return 1;
}
return 0;
}
/*
0 成功
1 失败
*/
int cmd_ext3_prs(int client, _MSG_NODE *msg)
{
u8_t sendbuf[1024];
int i, ret;
memset(sendbuf, 0, 1024);
get_format(sendbuf, msg->ctag);
sprintf((char *)sendbuf, "%s%c%c%c\"", sendbuf, SP, SP, SP);
for(i = 0; i < 10; i++)
{
if( (EXT_OUT16 == gExtCtx.extBid[2][i]) ||
(EXT_OUT32 == gExtCtx.extBid[2][i]) ||
(EXT_OUT16S == gExtCtx.extBid[2][i]) ||
(EXT_OUT32S == gExtCtx.extBid[2][i]) )
{
sprintf((char *)sendbuf, "%s%c,%s",
sendbuf,
gExtCtx.extBid[2][i],
((0 == strlen(gExtCtx.out[20 + i].outPR)) ? (char *)"," : (char *)(gExtCtx.out[20 + i].outPR)));
}
else
{
sprintf((char *)sendbuf, "%s%c,%s",
sendbuf,
gExtCtx.extBid[2][i],
",");
}
if(i < 9)
{
strcat(sendbuf, ":");
}
}
strcat(sendbuf, "\"\r\n;");
ret = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(ret == -1 && errno != EAGAIN)
{
return 1;
}
return 0;
}
/*
0 成功
1 失败
*/
int cmd_drv_mgr(int client, _MSG_NODE *msg)
{
u8_t sendbuf[1024];
int ret;
unsigned char drvPr[2][3];
unsigned char mgrPr[6];
memset(drvPr, 0, sizeof(drvPr));
memset(mgrPr, 0, sizeof(mgrPr));
memset(sendbuf, 0, 1024);
drvPr[0][0] = gExtCtx.drv[0].drvPR[0];
drvPr[1][0] = gExtCtx.drv[1].drvPR[0];
drvPr[0][1] = gExtCtx.drv[0].drvPR[1];
drvPr[1][1] = gExtCtx.drv[1].drvPR[1];
drvPr[0][2] = gExtCtx.drv[0].drvPR[2];
drvPr[1][2] = gExtCtx.drv[1].drvPR[2];
mgrPr[0] = gExtCtx.mgr[0].mgrPR;
mgrPr[1] = gExtCtx.mgr[1].mgrPR;
mgrPr[2] = gExtCtx.mgr[2].mgrPR;
mgrPr[3] = gExtCtx.mgr[3].mgrPR;
mgrPr[4] = gExtCtx.mgr[4].mgrPR;
mgrPr[5] = gExtCtx.mgr[5].mgrPR;
get_format(sendbuf, msg->ctag);
if( (((alm_sta[12][5] & 0x01) == 0x01) && (slot_type[12] == 'd') )
|| ( ((alm_sta[13][5] & 0x01) == 0x01) && (slot_type[13] == 'd') )
|| ((gExtCtx.save.onlineSta & 0x01) == 0))
{
drvPr[0][0] = 4;
drvPr[1][0] = 4;
mgrPr[0] = 4;
mgrPr[1] = 4;
}
if( (((alm_sta[12][5] & 0x02) == 0x02) && (slot_type[12] == 'd'))
|| ( ((alm_sta[13][5] & 0x02) == 0x02) && (slot_type[13] == 'd') )
|| ((gExtCtx.save.onlineSta & 0x02) == 0x00))
{
drvPr[0][1] = 4;
drvPr[1][1] = 4;
mgrPr[2] = 4;
mgrPr[3] = 4;
}
if( (((alm_sta[12][5] & 0x04) == 0x04) && (slot_type[12] == 'd'))
|| ( ((alm_sta[13][5] & 0x04) == 0x04) && (slot_type[13] == 'd') )
|| ((gExtCtx.save.onlineSta & 0x04) == 0x00))
{
drvPr[0][2] = 4;
drvPr[1][2] = 4;
mgrPr[4] = 4;
mgrPr[5] = 4;
}
sprintf((char *)sendbuf, "%s \"%d%d%d%d%d%d,%d%d%d%d%d%d\"\r\n;",
sendbuf,
drvPr[0][0], drvPr[1][0],
drvPr[0][1], drvPr[1][1],
drvPr[0][2], drvPr[1][2],
mgrPr[0], mgrPr[1],
mgrPr[2], mgrPr[3],
mgrPr[4], mgrPr[5] );
/*
sprintf((char *)sendbuf, "%s \"%d%d%d%d%d%d,%d%d%d%d%d%d\"\r\n;",
sendbuf,
gExtCtx.drv[0].drvPR[0], gExtCtx.drv[1].drvPR[0],
gExtCtx.drv[0].drvPR[1], gExtCtx.drv[1].drvPR[1],
gExtCtx.drv[0].drvPR[2], gExtCtx.drv[1].drvPR[2],
gExtCtx.mgr[0].mgrPR, gExtCtx.mgr[1].mgrPR,
gExtCtx.mgr[2].mgrPR, gExtCtx.mgr[3].mgrPR,
gExtCtx.mgr[4].mgrPR, gExtCtx.mgr[5].mgrPR );
*/
ret = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(ret == -1 && errno != EAGAIN)
{
return 1;
}
return 0;
}
int cmd_rtrv_out(int client , _MSG_NODE *msg )
{
unsigned char sendbuf[1024];
int send_ok, i;
unsigned char real_rpt;
memset(sendbuf, '\0', 1024);
get_format(sendbuf, msg->ctag);
sprintf((char *)sendbuf, "%s%c%c%c\"", sendbuf, SP, SP, SP);
for(i = 0; i < 14; i++)
{
if( (slot_type[i] == 'J') ||
(slot_type[i] == 'a' ) ||
(slot_type[i] == 'b') ||
(slot_type[i] == 'c') ||
(slot_type[i] == 'h') ||
(slot_type[i] == 'i') )
{
if('0' == rpt_content.slot[i].out_mode)
{
real_rpt = '2';
}
else
{
real_rpt = rpt_content.slot[i].out_sta;
}
sprintf((char *)sendbuf, "%s%c%c", sendbuf, slot_type[i], real_rpt);
}
if(i < 13)
{
strncatnew(sendbuf, ",", 1);
}
}
sprintf((char *)sendbuf, "%s\"%c%c;", sendbuf, CR, LF);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(send_ok == -1 && errno == EAGAIN)
{
/* close(client);
FD_CLR(client, &allset);
client= -1;*/
printf("timeout_<4>!\n");
}
return 0;
}
int cmd_set_CONFIG(_MSG_NODE *msg, int flag)
{
unsigned char buf[15] = "SET-CONFIG:::;";
if(file_exists(CONFIG_FILE))
{
printf("CONFIG wait\n");
sendtodown_cli(buf, msg->solt, msg->ctag);
}
else
{
return 1;
}
return 0;
}
int cmd_rtrv_eqpt(int client , _MSG_NODE *msg )
{
int send_ok;
unsigned char sendbuf[1024];
memset(sendbuf, '\0', sizeof(unsigned char) * 1024);
get_format(sendbuf, msg->ctag);
get_data(msg->solt, sendbuf);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
//printf("\n\n%s SEND: %s\n\n", __func__, sendbuf);
if(send_ok == -1 && errno == EAGAIN)
{
/* close(client);
FD_CLR(client, &allset);
client= -1;*/
printf("timeout_<4>!\n");
}
return 0;
}
/*
0 成功
1 失败
*/
int cmd_ext1_eqt(int client, _MSG_NODE *msg)
{
int ret;
u8_t sendbuf[1024];
memset(sendbuf, 0, 1024);
get_format(sendbuf, msg->ctag);
get_ext1_eqt(msg->solt, sendbuf);
ret = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(ret == -1 && errno != EAGAIN)
{
return 1;
}
return 0;
}
/*
0 成功
1 失败
*/
int cmd_ext2_eqt(int client, _MSG_NODE *msg)
{
int ret;
u8_t sendbuf[1024];
memset(sendbuf, 0, 1024);
get_format(sendbuf, msg->ctag);
get_ext2_eqt(msg->solt, sendbuf);
ret = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(ret == -1 && errno != EAGAIN)
{
return 1;
}
return 0;
}
/*
0 成功
1 失败
*/
int cmd_ext3_eqt(int client, _MSG_NODE *msg)
{
int ret;
u8_t sendbuf[1024];
memset(sendbuf, 0, 1024);
get_format(sendbuf, msg->ctag);
get_ext3_eqt(msg->solt, sendbuf);
ret = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(ret == -1 && errno != EAGAIN)
{
return 1;
}
return 0;
}
/*
0 成功
1 失败
*/
int cmd_ext_eqt(int client, _MSG_NODE *msg)
{
int ret;
u8_t sendbuf[1024];
memset(sendbuf, 0, 1024);
get_format(sendbuf, msg->ctag);
get_exta1_eqt(msg->solt, sendbuf);
get_exta2_eqt(msg->solt, sendbuf);
get_exta3_eqt(msg->solt, sendbuf);
ret = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(ret == -1 && errno != EAGAIN)
{
return 1;
}
return 0;
}
int cmd_rtrv_pm_inp(int client, _MSG_NODE *msg )
{
//unsigned char *sendbuf=(unsigned char *) malloc ( sizeof(unsigned char) * 1536);
unsigned char sendbuf[1536];
int i, send_ok;
memset(sendbuf, '\0', sizeof(unsigned char) * 1536);
get_format(sendbuf, msg->ctag);
/* <cr><lf><lf> ^^^<sid>^<date>^<time><cr><lf>
M^^<catg>^COMPLD<cr><lf>
[^^^<response message><cr><lf>]*
;*/
sprintf((char *)sendbuf, "%s%c%c%c\"", sendbuf, SP, SP, SP);
if(msg->solt == RB1_SLOT)
{
for(i = 0; i < 10; i++)
{
strncatnew(sendbuf, rpt_content.slot_o.rb_inp[i], strlen(rpt_content.slot_o.rb_inp[i]));
}
}
else if(msg->solt == RB2_SLOT)
{
for(i = 0; i < 10; i++)
{
strncatnew(sendbuf, rpt_content.slot_p.rb_inp[i], strlen(rpt_content.slot_p.rb_inp[i]));
}
}
sprintf((char *)sendbuf, "%s\"%c%c;", sendbuf, CR, LF);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(send_ok == -1 && errno == EAGAIN)
{
/* close(client);
FD_CLR(client, &allset);
client= -1;*/
printf("timeout_<5>!\n");
}
// free(sendbuf);
// sendbuf=NULL;
return 0;
}
int cmd_rtrv_pm_msg(int client , _MSG_NODE *msg )
{
/*unsigned char *sendbuf=(unsigned char *) malloc ( sizeof(unsigned char) * SENDBUFSIZE);
memset(sendbuf,'\0',SENDBUFSIZE);
free(sendbuf);*/
/*"[<aid>:<type>,<date>,<time>,<almcde>,<ctfcncde>,<condtype>,<serveff>;"<cr><lf>]*"*/
//unsigned char *sendbuf=(unsigned char *) malloc ( sizeof(unsigned char) * 3072);
unsigned char sendbuf[3072];
int i, send_ok, len;
memset(sendbuf, '\0', sizeof(unsigned char) * 3072);
get_format(sendbuf, msg->ctag);
/* <cr><lf><lf> ^^^<sid>^<date>^<time><cr><lf>
M^^<catg>^COMPLD<cr><lf>
[^^^<response message><cr><lf>]*
;*/
sprintf((char *)sendbuf, "%s%c%c%c\"", sendbuf, SP, SP, SP);
for(i = 0; i < SAVE_ALM_NUM; i++)
{
len = strlen(saved_alms[i]);
if(len > 0)
{
strncatnew(sendbuf, saved_alms[i], len);
}
}
sprintf((char *)sendbuf, "%s\"%c%c;", sendbuf, CR, LF);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(send_ok == -1 && errno == EAGAIN)
{
/* close(client);
FD_CLR(client, &allset);
client= -1;*/
printf("timeout_<5>!\n");
}
// free(sendbuf);
// sendbuf=NULL;
return 0;
}
int cmd_set_ref_prio(_MSG_NODE *msg, int flag)
{
int num, i, len;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
len = strlen(msg->data);
num = (int)(msg->solt - 0x61);
if((num < 0) || (num > 13))
{
return 1;
}
if(len == 9)
{
if(msg->data[0] == ',') //wu prio1
{
for(i = 0; i < 8; i++)
{
if((msg->data[i + 1] < 0x30) || (msg->data[i + 1] > 0x38))
{
return 1;
}
}
memcpy(conf_content.slot[num].ref_prio2, &msg->data[1], 8);
}
else
{
for(i = 0; i < 8; i++)
{
if((msg->data[i] < 0x30) || (msg->data[i] > 0x38))
{
return 1;
}
}
memcpy(conf_content.slot[num].ref_prio1, msg->data, 8);
}
}
else if(len == 17)
{
for(i = 0; i < 8; i++)
{
if((msg->data[i + 9] < 0x30) || (msg->data[i + 9] > 0x38))
{
return 1;
}
if((msg->data[i] < 0x30) || (msg->data[i] > 0x38))
{
return 1;
}
}
memcpy(conf_content.slot[num].ref_prio2, &msg->data[9], 8);
memcpy(conf_content.slot[num].ref_prio1, msg->data, 8);
}
else
{
return 1;
}
sprintf((char *)sendbuf, "SET-REF-PRIO:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ref_mod(_MSG_NODE *msg, int flag)
{
int num, len;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
len = strlen(msg->data);
num = (int)(msg->solt - 0x61);
if((num < 0) || (num > 13))
{
return 1;
}
if(len == 5)
{
if(msg->data[0] == ',')
{
if(((msg->data[2] == '0') || (msg->data[2] == '1')) &&
((msg->data[4] > 0x2f) && (msg->data[4] < 0x39)))
{
memcpy(conf_content.slot[num].ref_mod2, &msg->data[2], 3);
}
else
{
return 1;
}
}
else
{
if(((msg->data[0] == '0') || (msg->data[0] == '1')) &&
((msg->data[2] > 0x2f) && (msg->data[2] < 0x39)))
{
memcpy(conf_content.slot[num].ref_mod1, &msg->data[0], 3);
}
else
{
return 1;
}
}
}
else if(len == 7)
{
if(((msg->data[0] == '0') || (msg->data[0] == '1')) &&
((msg->data[2] > 0x2f) && (msg->data[2] < 0x39)))
{}
else
{
return 1;
}
if(((msg->data[4] == '0') || (msg->data[4] == '1')) &&
((msg->data[6] > 0x2f) && (msg->data[6] < 0x39)))
{}
else
{
return 1;
}
memcpy(conf_content.slot[num].ref_mod1, &msg->data[0], 3);
memcpy(conf_content.slot[num].ref_mod2, &msg->data[4], 3);
}
else
{
return 1;
}
sprintf((char *)sendbuf, "SET-REF-MOD:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ref_sa(_MSG_NODE *msg, int flag)
{
int i;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
for(i = 0; i < 8; i++)
{
if((msg->data[i] < '@') && (msg->data[i] > 'E'))
{
return 1;
}
}
sprintf((char *)sendbuf, "SET-REF-SA:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ref_tlb(_MSG_NODE *msg, int flag)
{
int i;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
for(i = 0; i < 8; i++)
{
if((msg->data[i] < 'a') || (msg->data[i] > 'g'))
{
return 1;
}
}
sprintf((char *)sendbuf, "SET-REF-TLB:%s::%s;", msg->ctag, msg->data);
//printf("%s\n","BBBB");
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ref_tlm(_MSG_NODE *msg, int flag)
{
int num;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
num = (int)(msg->solt - 0x61);
if((msg->data[0] != '0') && (msg->data[0] != '1'))
{
return 1;
}
sprintf((char *)sendbuf, "SET-REF-TLM:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
conf_content.slot[num].ref_2mb_lvlm = msg->data[0];
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ref_tl(_MSG_NODE *msg, int flag)
{
int i;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
for(i = 0; i < 8; i++)
{
if((msg->data[i] < 'a') && (msg->data[i] > 'g'))
{
return 1;
}
}
sprintf((char *)sendbuf, "SET-REF-TL:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ref_ssm_en(_MSG_NODE *msg, int flag)
{
int num, i;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
num = (int)(msg->solt - 0x61);
for(i = 0; i < 8; i++)
{
if((msg->data[i] < '0') && (msg->data[i] > '2'))
{
return 1;
}
}
sprintf((char *)sendbuf, "SET-REF-IEN:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
memcpy(conf_content.slot[num].ref_in_ssm_en, &msg->data[0], 8);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ref_ph(_MSG_NODE *msg, int flag)
{
int i;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
for(i = 0; i < 8; i++)
{
if((msg->data[i] != '0') && (msg->data[i] != '1'))
{
return 1;
}
}
sprintf((char *)sendbuf, "SET-REF-PH:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_rs_en(_MSG_NODE *msg, int flag)
{
int num, i;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
for(i = 0; i < 4; i++)
{
if((msg->data[i] != '0') && (msg->data[i] != '1'))
{
return 1;
}
}
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
num = (int)(msg->solt - 0x61);
memcpy(conf_content.slot[num].rs_en, msg->data, 4);
sprintf((char *)sendbuf, "SET-RS-EN:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
else
{
return 1;
}
return 0;
}
int cmd_set_rs_tzo(_MSG_NODE *msg, int flag)
{
int num, i;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
for(i = 0; i < 4; i++)
{
if((msg->data[i] < 0x40) || (msg->data[i] > 0x57))
{
return 1;
}
}
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
num = (int)(msg->solt - 0x61);
memcpy(conf_content.slot[num].rs_tzo, msg->data, 4);
sprintf((char *)sendbuf, "SET-RS-TZO:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
else
{
return 1;
}
return 0;
}
int cmd_set_ppx_mod(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num = 0, i;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
for(i = 0; i < 16; i++)
{
if((msg->data[i] != '1') && (msg->data[i] != '2') && (msg->data[i] != '3'))
{
return 1;
}
}
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
num = (int)(msg->solt - 0x61);
memcpy(conf_content.slot[num].ppx_mod, msg->data, 16);
sprintf((char *)sendbuf, "SET-PPX-MOD:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
else
{
return 1;
}
return 0;
}
int cmd_set_tod_en(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num = 0, i;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
for(i = 0; i < 16; i++)
{
if((msg->data[i] != '1') && (msg->data[i] != '0'))
{
return 1;
}
}
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
num = (int)(msg->solt - 0x61);
memcpy(conf_content.slot[num].tod_en, msg->data, 16);
sprintf((char *)sendbuf, "SET-TOD-EN:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
else
{
return 1;
}
return 0;
}
int cmd_set_tod_pro(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num = 0;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
num = (int)(msg->solt - 0x61);
// memcpy(conf_content.slot[num].tod_en, msg->data, 16);
if(msg->data[0] == ',')
{
if((msg->data[1] < 0x40) || (msg->data[1] > 0x57))
{
return 1;
}
else
{
conf_content.slot[num].tod_tzo = msg->data[1];
}
}
else
{
if((msg->data[0] < 0x30) || (msg->data[0] > 0x37))
{
return 1;
}
else
{
if(msg->data[1] == ',')
{
if( (msg->data[2] < 0x40) || (msg->data[2] > 0x57) )
{
//return 1;
}
else
{
conf_content.slot[num].tod_tzo = msg->data[2];
}
}
else
{
return 1;
}
}
conf_content.slot[num].tod_br = msg->data[0];
}
sprintf((char *)sendbuf, "SET-TOD-PRO:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
return 0;
}
int cmd_set_igb_en(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num = 0, i;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
for(i = 0; i < 4; i++)
{
if((msg->data[i] != '1') && (msg->data[i] != '0'))
{
return 1;
}
}
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
num = (int)(msg->solt - 0x61);
memcpy(conf_content.slot[num].igb_en, msg->data, 4);
sprintf((char *)sendbuf, "SET-IGB-EN:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
else
{
return 1;
}
return 0;
}
int cmd_set_igb_rat(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num = 0, i;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
for(i = 0; i < 4; i++)
{
if((msg->data[i] < 'a') && (msg->data[i] > 'i'))
{
return 1;
}
}
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
num = (int)(msg->solt - 0x61);
memcpy(conf_content.slot[num].igb_rat, msg->data, 4);
sprintf((char *)sendbuf, "SET-IGB-RAT:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
else
{
return 1;
}
return 0;
}
int cmd_set_igb_max(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num = 0, i;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
for(i = 0; i < 4; i++)
{
if((msg->data[i] < 'A') && (msg->data[i] > 'E'))
{
return 1;
}
}
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
num = (int)(msg->solt - 0x61);
memcpy(conf_content.slot[num].igb_max, msg->data, 4);
sprintf((char *)sendbuf, "SET-IGB-MAX:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
else
{
return 1;
}
return 0;
}
int cmd_set_ntp_net(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num = 0, i, len = 0;
int j, i1, i2, i3;
j = i1 = i2 = i3 = 0;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
num = (int)(msg->solt - 0x61);
len = strlen(msg->data);
for(i = 0; i < len; i++)
{
if(msg->data[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
}
}
if(i3 == 0)
{
return 1;
}
if(msg->data[0] != ',')
{
memcpy(conf_content.slot[num].ntp_ip, &msg->data[0], i1 - 1);
}
if(msg->data[i1] != ',')
{
memcpy(conf_content.slot[num].ntp_mac, &msg->data[i1], i2 - i1 - 1);
}
if(msg->data[i2] != ',')
{
memcpy(conf_content.slot[num].ntp_gate, &msg->data[i2], i3 - i2 - 1);
}
if(msg->data[i3] != '\0')
{
memcpy(conf_content.slot[num].ntp_msk, &msg->data[i3], len - i3);
}
sprintf((char *)sendbuf, "SET-NTP-NET:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ntp_pnet(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int len = 0;
int num;
memset(sendbuf,0, sizeof(unsigned char) * SENDBUFSIZE);
len = strlen(msg->data);
num = (int)(msg->solt - 0x61);
sprintf((char *)sendbuf, "SET-NTP-PNET:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ntp_pmac(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int len = 0;
memset(sendbuf,0, sizeof(unsigned char) * SENDBUFSIZE);
len = strlen(msg->data);
sprintf((char *)sendbuf, "SET-NTP-PMAC:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ntp_pen(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int len = 0;
memset(sendbuf,0, sizeof(unsigned char) * SENDBUFSIZE);
len = strlen(msg->data);
sprintf((char *)sendbuf, "SET-NTP-PEN:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ntp_en(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num = 0, i, len;
int j, i1, i2, i3, i4;
j = i1 = i2 = i3 = i4 = 0;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
num = (int)(msg->solt - 0x61);
len = strlen(msg->data);
for(i = 0; i < len; i++)
{
if(msg->data[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
}
}
#if 1
if((msg->data[0] == '0') || (msg->data[0] == '1'))
{
conf_content.slot[num].ntp_bcast_en = msg->data[0];
}
if(msg->data[i1] != ',')
{
memcpy(conf_content.slot[num].ntp_interval, &msg->data[i1], i2 - i1 - 1);
}
if((msg->data[i2] > 0x40) && (msg->data[i2] < 0x50))
{
conf_content.slot[num].ntp_tpv_en = msg->data[i2];
}
if((msg->data[i3] == '0') || (msg->data[i3] == '1'))
{
conf_content.slot[num].ntp_md5_en = msg->data[i3];
}
/*if((msg->data[i4] == '0') || (msg->data[i4] == '1'))
{
conf_content.slot[num].ntp_ois_en = msg->data[i4];
}*/
#endif
sprintf((char *)sendbuf, "SET-NTP-EN:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ntp_key(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
unsigned char keyid[5];
unsigned char i =0;
int num = 0;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
memset(keyid, '\0', 5);
num = (int)(msg->solt - 0x61);
if((msg->data[0] != '0') && (msg->data[0] != '1') && (msg->data[0] != '2'))
{
return 1;
}
else if(msg->data[1] != ',')
{
return 1;
}
else if(msg->data[6] != ',')
{
return 1;
}
#if 1
memcpy(keyid, &msg->data[2], 4);
if(msg->data[0]=='0')
{
for(i=0;i<4;i++)
{
if(conf_content.slot[num].ntp_key_flag[i]!=2)
{
conf_content.slot[num].ntp_key_flag[i]=2;
memcpy(conf_content.slot[num].ntp_keyid[i], &msg->data[2], 4);
memcpy(conf_content.slot[num].ntp_key[i], &msg->data[7], 16);
break;
}
//else if(i==3)
// return 1;
}
}
else if(msg->data[0]=='1')
{
for(i=0;i<4;i++)
{
if(strncmp1(keyid, conf_content.slot[num].ntp_keyid[i] , 4)==0)
{
conf_content.slot[num].ntp_key_flag[i]=1;
memset(conf_content.slot[num].ntp_keyid[i],'\0',5);
memset(conf_content.slot[num].ntp_key[i],'\0',17);
break;
}
//else if(i==3)
// return 1;
}
}
else if(msg->data[0]=='2')
{
for(i=0;i<4;i++)
{
if(strncmp1(keyid, conf_content.slot[num].ntp_keyid[i] , 4)==0)
{
memset(conf_content.slot[num].ntp_key[i],'\0',17);
memcpy(conf_content.slot[num].ntp_key[i], &msg->data[7], 16);
break;
}
//else if(i==3)
// return 1;
}
}
#endif
sprintf((char *)sendbuf, "SET-NTP-KEY:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_ntp_ms(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-NTP-MS:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
//save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_mcp_fb(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
if((msg->data[0] != '0') && (msg->data[0] != '1'))
{
return 1;
}
if(conf_content.slot_u.fb == msg->data[0])
{}
else
{
conf_content.slot_u.fb = msg->data[0];
sprintf((char *)sendbuf, "SET-RB-FB:%s::%s;", msg->ctag, msg->data);
if(flag)
{
if(slot_type[14] == 'R' || slot_type[14] == 'K')
{
sendtodown_cli(sendbuf, RB1_SLOT, msg->ctag);
}
if(slot_type[15] == 'R' || slot_type[15] == 'K')
{
sendtodown_cli(sendbuf, RB2_SLOT, msg->ctag);
}
}
else
//sendtodown(sendbuf, msg->solt);
{
if(slot_type[14] == 'R' || slot_type[14] == 'K')
{
sendtodown(sendbuf, RB1_SLOT);
}
if(slot_type[15] == 'R' || slot_type[15] == 'K')
{
sendtodown(sendbuf, RB2_SLOT);
}
}
save_config();
}
respond_success(current_client, msg->ctag);
return 0;
}
int cmd_set_rb_sa(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
if((msg->data[0] < '@') || (msg->data[0] > 'E'))
{
return 1;
}
sprintf((char *)sendbuf, "SET-RB-SA:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
//save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
int cmd_set_rb_tl(_MSG_NODE *msg, int flag)
{
if((msg->data[0] < 'a') || (msg->data[0] > 'g'))
{
return 1;
}
if(msg->solt == RB1_SLOT)
{
rpt_content.slot_o.rb_tl_2mhz = msg->data[0];
}
else if(msg->solt == RB2_SLOT)
{
rpt_content.slot_p.rb_tl_2mhz = msg->data[0];
}
get_out_lev(0, 0);
respond_success(current_client, msg->ctag);
return 0;
}
//
//!设置钟盘1槽位REFTF输入IRIGB信号性能超限阀值
//!设置钟盘2槽位REFTF输入IRIGB信号性能超限阀值
//
int cmd_set_rb_thresh(_MSG_NODE *msg, int flag)
{
int len, num;
int temp;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
len = strlen(msg->data);
if((msg->data[0] < 0x31)&&(msg->data[0] > 0x32)) //参考输入IRIGB信号的槽位号{1:2}
{
return 1;
}
temp = atoi(&msg->data[2]);
if((temp < 1) || (temp > 999999))//6字节,最大为999,999,最小为1
{
return 1;
}
if(msg->solt == RB1_SLOT) //钟盘槽位号15+0x60
{
num = (int)(msg->data[0] - 0x31);//参考输入IRIGB信号的槽位号{1:2}
if((num >= 0x00) && (num <= 0x01))
{
//if(strcmp(conf_content.slot_o.dely[num], &msg->data[2])!=0)
// {
memset(conf_content.slot_o.thresh[num], '\0', 7);
memcpy(conf_content.slot_o.thresh[num], &msg->data[2], len - 2);
sprintf((char *)sendbuf, "SET-RB-TH:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
//}
}
}
else if(msg->solt == RB2_SLOT) //钟盘槽位号16+0x60
{
num = (int)(msg->data[0] - 0x31); //参考输入IRIGB信号的槽位号{1:2}
if((num >= 0x00) && (num <= 0x01))
{
//if(strcmp(rpt_content.slot_p., &msg->data[2])!=0)
// {
memset(conf_content.slot_p.thresh[num], '\0', 7);
memcpy(conf_content.slot_p.thresh[num], &msg->data[2], len - 2);
printf("set rb thresh%s,%s \n",conf_content.slot_p.thresh[0],conf_content.slot_p.thresh[1]);
sprintf((char *)sendbuf, "SET-RB-TH:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
// }
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
int cmd_set_alm_msk(_MSG_NODE *msg, int inflag)
{
//msg->data:[<type>,<xxxxxxxxxxx>]+
int typeflag = 0; //1:表示为msg->data的type段;0:表示为msg->data的xxxxxxxxxxx段;
int num;
int i, j, len;
int msgType[128], index = 0;
unsigned char *data;
unsigned char errflag;
unsigned char maxlen;
// downflag[3] [0]:REFTF [1]:RB 2MHZ/2MB [2]GBTP
//unsigned char almmsknum[3];
//unsigned char sendbuf[SENDBUFSIZE];
//unsigned char maskdata[17];
//unsigned char tmpdata[7];
errflag = 0;
maxlen = 0;
//memset(tmpdata,'\0',7);
//memset(maskdata,'\0',17);
//memset(sendbuf, '\0', SENDBUFSIZE);
//memset(almmsknum,'\0',3);
printf("-----\n%s:\n", __FUNCTION__);
if(NULL == msg)
{
printf("cmd_set_alm_msk is error\n");
return -1;
}
else
{
len = strlen(msg->data);
data = (char *)malloc(len);
if(NULL == data)
{
printf("cmd_set_alm_msk Malloc is failed\n");
return -1;
}
else
{
memset(data, 0, len);
memcpy(data, msg->data, len);
printf("Msk data:%s\n", data);
}
}
for(i = 0; i < len; i++)
{
if('=' == data[i])
{
msgType[index] = i - 1; //msg->data的type段所在位置
index++;
}
}
//
//!the tail of msgtype array mark as -1. the -1 mean terminiter.
msgType[index] = -1;
index = 0;
i = 0;
num = -1;
//
//! Check the MsgType is or not out of bounds.
//
while(-1 != msgType[index])
{
//
//if the i equal to the value of the msgType,find the table of mask .
//
if(i == msgType[index])
{
typeflag = 1;
errflag = 1;
}
else
{
typeflag = 0;
}
//
// The Header Msgtype Should Be Corrected,or not the fuc don't deal.
//
if(1 == errflag)
{
if(1 == typeflag)
{
printf("The CMD RALM----slot_type:%c\n", data[i]);
for(j = 0; j < alm_msk_total; j++)
{
//if equal S or K, record the num of mask.
//almmsknum[0] = (data[i] == 'S')? j : 0;
//almmsknum[1] = (data[i] == 'K')? j : 0;
if(data[i] == conf_content.alm_msk[j].type)
{
num = j;
break;
}
}
if(-1 == num)
{
for(j = 0; j < alm_msk_total; j++)
{
if('\0' == conf_content.alm_msk[j].type)
{
conf_content.alm_msk[j].type = data[i];
num = j;
break;
}
}
}
i += 2;
}
else
{
printf("num= %d\n", num);
index++;
if(-1 != msgType[index])
{
//
//limit the max len of data to 6。
//
maxlen = msgType[index] - i - 1;
if(maxlen <= ALM_MASK_LEN)
{
if((data[i] >> 4) == 0x4)
{
memcpy(conf_content.alm_msk[num].data, &data[i], msgType[index] - i - 1);
i = msgType[index];
}
else
{
memcpy(conf_content.alm_msk[num].data + 6, &data[i], msgType[index] - i - 1);
i = msgType[index];
}
}
printf("-1 != msgType[index]---%c:%s\n", conf_content.alm_msk[num].type, conf_content.alm_msk[num].data);
}
else
{
//
//limit the max len of data to 6。
//
maxlen = len - i;
if(maxlen <= ALM_MASK_LEN)
{
if((data[i] >> 4) == 0x4)
{
memcpy(conf_content.alm_msk[num].data, &data[i], len - i);
}
else
{
memcpy(conf_content.alm_msk[num].data + 6, &data[i], len - i);
}
}
//printf("-1 == msgType[index]---%c %s\n",conf_content.alm_msk[num].type,conf_content.alm_msk[num].data);
}
typeflag = 1;
num = -1;
}
}
else
{
msgType[index] = -1;
}
}
free(data);
//
//REFTF E1 OR TIME PORT LAMP ON/OFF control;
/*if(almmsknum[0])
{
num = almmsknum[0];
tmpdata[2] = (conf_content.alm_msk[num].data[3] & 0x0C) >> 2;
tmpdata[2] |= (conf_content.alm_msk[num].data[2] & 0x03) <<2 ;
tmpdata[3] = (conf_content.alm_msk[num].data[2] & 0x0C) >>2;
tmpdata[3] |= ( conf_content.alm_msk[num].data[3] & 0x03) <<2;
tmpdata[0] = conf_content.alm_msk[num].data[4] &0x0f;
tmpdata[1] = conf_content.alm_msk[num].data[5] &0x0f;
for(i = 0; i < 4;i++)
for(j = 0; j < 4; j++)
{
//
if((tmpdata[i] & (1 << j)) == 0)
maskdata[4*i+j] = '0';
else
maskdata[4*i+j] = '1';
}
for(i = 0; i <14; i++)
{
if(slot_type[i] == 'S')
break;
}
sprintf((char *)sendbuf, "SET-REF-LAMP:%s::%s;", msg->ctag, maskdata);
sendtodown(sendbuf, i + 0x61);
}*/
save_config();
return 0;
}
//cmd_rtrv_ralm_mask
int cmd_rtrv_ralm_mask(int client , _MSG_NODE *msg )
{
unsigned char sendbuf[3072];
int i, send_ok, len;
memset(sendbuf, '\0', sizeof(unsigned char) * 3072);
get_format(sendbuf, msg->ctag);
/* <cr><lf><lf> ^^^<sid>^<date>^<time><cr><lf>
M^^<catg>^COMPLD<cr><lf>
[^^^<response message><cr><lf>]*
;*/
/*[<type>:<data>:<cr><lf>]*/
sprintf((char *)sendbuf, "%s%c%c%c\"", sendbuf, SP, SP, SP);
for(i = 0; i < alm_msk_total; i++)
{
if(conf_content.alm_msk[i].type == '\0')
{
len = strlen(sendbuf);
if(',' == sendbuf[len - 1])
{
sendbuf[len - 1] = '\0';
}
break;
}
//out盘等输出盘不上传
else if(conf_content.alm_msk[i].type == 'B' ||
conf_content.alm_msk[i].type == 'D' ||
conf_content.alm_msk[i].type == 'E' ||
conf_content.alm_msk[i].type == 'F' ||
conf_content.alm_msk[i].type == 'G' ||
conf_content.alm_msk[i].type == 'H' ||
conf_content.alm_msk[i].type == 'J' ||
conf_content.alm_msk[i].type == 'a' ||
conf_content.alm_msk[i].type == 'c' ||
conf_content.alm_msk[i].type == 'b' ||
//conf_content.alm_msk[i].type == 'f' ||
conf_content.alm_msk[i].type == 'h' ||
conf_content.alm_msk[i].type == 'i'
)
{
continue;
}
if( (conf_content.alm_msk[i].data[6] >> 4) == 0x5 )
{
sprintf((char *)sendbuf, "%s%c%c", sendbuf, conf_content.alm_msk[i].type, '=');
strncatnew(sendbuf, conf_content.alm_msk[i].data, 6);
strncatnew(sendbuf, ":", 1);
strncatnew(sendbuf, &(conf_content.alm_msk[i].data[6]), 6);
}
else
{
sprintf((char *)sendbuf, "%s%c%c%s", sendbuf, conf_content.alm_msk[i].type, '=', conf_content.alm_msk[i].data);
}
if(conf_content.alm_msk[i + 1].type != '\0' && i + 1 < alm_msk_total)
{
strncatnew(sendbuf, ",", 1);
}
else
{
//sprintf((char *)sendbuf,"%s\"%c%c;",sendbuf,CR,LF);
}
}
sprintf((char *)sendbuf, "%s\"%c%c;", sendbuf, CR, LF);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(send_ok == -1 && errno == EAGAIN)
{
printf("timeout_<5>!\n");
}
return 0;
}
int cmd_set_ext_online(_MSG_NODE *msg, int flag)
{
//
//!
//
unsigned char onlineSta = '\0';
int i;
if(msg == NULL)
{
return 1;
}
onlineSta = msg->data[0];
for(i = 0; i < 3; i++)
{
if( ( (onlineSta >> i) & 0x01 ) == 0 )
{
memset(gExtCtx.extBid[i], 'O', 15);
}
else
{
;
}
}
gExtCtx.save.onlineSta = onlineSta;
if(0 == ext_write_flash(&(gExtCtx.save)))
{
return 1;
}
return 0;
}
int cmd_qext_online(int client, _MSG_NODE *msg)
{
int send_ok, num;
unsigned char sendbuf[1024];
memset(sendbuf, '\0', sizeof(unsigned char) * 1024);
num = (int)(msg->solt - 'a');
get_format(sendbuf, msg->ctag);
sprintf((char *)sendbuf, "%s%c%c%c\"", sendbuf, SP, SP, SP);
if( (gExtCtx.save.onlineSta & 0xF0) != 0x40 )
{
gExtCtx.save.onlineSta = 0x47;
sprintf((char *)sendbuf, "%s%c", sendbuf, gExtCtx.save.onlineSta);
}
else
{
sprintf((char *)sendbuf, "%s%c", sendbuf, gExtCtx.save.onlineSta);
}
sprintf((char *)sendbuf, "%s\"%c%c;", sendbuf, CR, LF);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(send_ok == -1 && errno == EAGAIN)
{
printf("timeout_<3>!\n");
}
return 0;
}
int cmd_set_leap_mod(_MSG_NODE *msg, int flag)
{
char leapMod;
leapMod = msg->data[0];
conf_content3.LeapMod = 0;
conf_content3.LeapMod = leapMod;
save_config();
return 0;
}
/*
2014.12.10
返回闰秒模式
0---手动
1---自动
*/
int cmd_rtrv_leap_mod(int client, _MSG_NODE *msg)
{
int send_ok, num;
unsigned char sendbuf[1024];
memset(sendbuf, '\0', sizeof(unsigned char) * 1024);
num = (int)(msg->solt - 'a');
get_format(sendbuf, msg->ctag);
sprintf((char *)sendbuf, "%s%c%c%c\"", sendbuf, SP, SP, SP);
if( '0' != conf_content3.LeapMod && '1' != conf_content3.LeapMod)
{
conf_content3.LeapMod = '0';
sprintf((char *)sendbuf, "%s%c", sendbuf, conf_content3.LeapMod);
}
else
{
sprintf((char *)sendbuf, "%s%c", sendbuf, conf_content3.LeapMod);
}
sprintf((char *)sendbuf, "%s\"%c%c;", sendbuf, CR, LF);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(send_ok == -1 && errno == EAGAIN)
{
printf("timeout_<3>!\n");
}
return 0;
}
int cmd_rtrv_igb_tzone(int client, _MSG_NODE *msg)
{
int send_ok, num;
unsigned char sendbuf[1024];
num = (int)(msg->solt - 'a');
if( ((msg->solt) <= '`') || ((msg->solt) >= 'o') )
{
return 1;
}
else if( slot_type[num] != 'W' )
{
return 1;
}
else
{
memset(sendbuf, '\0', sizeof(unsigned char) * 1024);
}
get_format(sendbuf, msg->ctag);
sprintf((char *)sendbuf, "%s%c%c%c\"", sendbuf, SP, SP, SP);
if( '0' == rpt_content.slot[num].igb_tzone || '8' == rpt_content.slot[num].igb_tzone)
{
sprintf((char *)sendbuf, "%s%c", sendbuf, rpt_content.slot[num].igb_tzone);
}
else
{
sprintf((char *)sendbuf, "%s%c", sendbuf, '0');
}
sprintf((char *)sendbuf, "%s\"%c%c;", sendbuf, CR, LF);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(send_ok == -1 && errno == EAGAIN)
{
printf("timeout_<3>!\n");
}
return 0;
}
/*
2015.5.27
set irigb16 timezone
*/
int cmd_set_igb_tzone(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num = 0;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
if( ((msg->solt) > '`') && ((msg->solt) < 'o') )
{
num = (int)(msg->solt - 0x61);
if(slot_type[num] != 'W')
{
return 1;
}
conf_content3.irigb_tzone[num] = (msg->data)[0];
sprintf((char *)sendbuf, "SET-IGB-TZONE:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
return 0;
}
else
{
return 1;
}
}
int cmd_sys_cpu(int client, _MSG_NODE *msg)
{
int send_ok;
unsigned char sendbuf[1024];
char *sys_cpu = "6";
char *sys_mem = "10";
memset(sendbuf, '\0', sizeof(unsigned char) * 1024);
get_format(sendbuf, msg->ctag);
sprintf((char *)sendbuf, "%s%c%c%c\"", sendbuf, SP, SP, SP);
sprintf((char *)sendbuf, "%s%s,%s", sendbuf, sys_cpu, sys_mem);
sprintf((char *)sendbuf, "%s\"%c%c;", sendbuf, CR, LF);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(send_ok == -1 && errno == EAGAIN)
{
printf("timeout_<3>!\n");
}
return 0;
}
int read_ip(unsigned const char *ip, unsigned char *out_ip)
{
//0100 Y7Y6Y5Y4 0100 Y3Y2Y1Y0
int i;
unsigned char temp = 0;
char in_ip[9];
if(in_ip == NULL || out_ip == NULL)
{
printf("read ip error\n");
return -1;
}
else if(*in_ip == ',')
{
return 1;
}
else
{
memset(in_ip,0,9);
memcpy(in_ip,ip,8);
}
for(i = 0; i < 8; i++)
{
if( ((*(in_ip + i)) & 0xf0) != 0x40 )
{
return 1;
}
else
{
if( (i & 0x01) == 0x00 )
{
temp = (*(in_ip + i)) << 4;
}
else
{
temp |= ((*(in_ip + i)) & 0x0f);
sprintf((char *)out_ip, "%s%d", out_ip, temp);
if(7 == i)
{
;
}
else
{
sprintf((char *)out_ip, "%s.", out_ip);
}
temp = 0;
}
}
}
return 0;
}
int cmd_rtrv_mtc_ip(int client, _MSG_NODE *msg)
{
int num, send_ok;
unsigned char sendbuf[1024];
unsigned char sendtmp[256];
memset(sendbuf, '\0', sizeof(unsigned char) * 1024);
memset(sendtmp, '\0', sizeof(unsigned char) * 256);
num = (int)(msg->solt - 'a');
get_format(sendtmp, msg->ctag);
sprintf((char *)sendbuf, "%s%c%c%c\"RPT-MTC-IP,%s,%s,%s,%s\"%c%c;",sendtmp,SP, SP, SP, &rpt_ptp_mtc.ptp_mtc_ip[num][0][0],
&rpt_ptp_mtc.ptp_mtc_ip[num][1][0],
&rpt_ptp_mtc.ptp_mtc_ip[num][2][0],
&rpt_ptp_mtc.ptp_mtc_ip[num][3][0],
CR,LF);
/*sprintf((char *)sendbuf, "%sRPT-MTC-IP,%s,%s,%s,%s", sendbuf,
&rpt_ptp_mtc.ptp_mtc_ip[num][0][0],
&rpt_ptp_mtc.ptp_mtc_ip[num][1][0],
&rpt_ptp_mtc.ptp_mtc_ip[num][2][0],
&rpt_ptp_mtc.ptp_mtc_ip[num][3][0]);*/
/*sprintf((char *)sendbuf, "%s\"%c%c", sendbuf, CR, LF);*/
//printf("mtc send %s\n",&rpt_ptp_mtc.ptp_mtc_ip[num][0][0]);
//printf("mtc send %d,%s\n",num,sendbuf);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(send_ok == -1 && errno == EAGAIN)
{
printf("timeout_<3>!\n");
}
return 0;
}
int cmd_rtrv_uni_ip(int client, _MSG_NODE *msg)
{
int num, send_ok;
unsigned char sendbuf[1024];
unsigned char sendtmp[256];
memset(sendbuf, '\0', sizeof(unsigned char) * 1024);
memset(sendtmp, '\0', sizeof(unsigned char) * 256);
num = (int)(msg->solt - 'a');
get_format(sendtmp, msg->ctag);
sprintf((char *)sendbuf, "%s%c%c%c\"RPT-MTC-IP,%s|%s|%s|%s\"%c%c;",sendtmp,SP, SP, SP, &rpt_ptp_mtc.ptp_mtc_ip[num][0][0],
&rpt_ptp_mtc.ptp_mtc_ip[num][1][0],
&rpt_ptp_mtc.ptp_mtc_ip[num][2][0],
&rpt_ptp_mtc.ptp_mtc_ip[num][3][0],
CR,LF);
/*sprintf((char *)sendbuf, "%sRPT-MTC-IP,%s,%s,%s,%s", sendbuf,
&rpt_ptp_mtc.ptp_mtc_ip[num][0][0],
&rpt_ptp_mtc.ptp_mtc_ip[num][1][0],
&rpt_ptp_mtc.ptp_mtc_ip[num][2][0],
&rpt_ptp_mtc.ptp_mtc_ip[num][3][0]);*/
/*sprintf((char *)sendbuf, "%s\"%c%c", sendbuf, CR, LF);*/
//printf("mtc send %s\n",&rpt_ptp_mtc.ptp_mtc_ip[num][0][0]);
//printf("mtc send %d,%s\n",num,sendbuf);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(send_ok == -1 && errno == EAGAIN)
{
printf("timeout_<3>!\n");
}
return 0;
}
int cmd_rtrv_mtc_dom(int client, _MSG_NODE *msg)
{
int num, send_ok;
unsigned char sendbuf[1024];
memset(sendbuf, '\0', sizeof(unsigned char) * 1024);
num = (int)(msg->solt - 'a');
get_format(sendbuf, msg->ctag);
sprintf((char *)sendbuf, "%sRPT-MTC-DOM,%s,%s,%s,%s;", sendbuf,
&rpt_ptp_mtc.ptp_dom[num][0][0],
&rpt_ptp_mtc.ptp_dom[num][1][0],
&rpt_ptp_mtc.ptp_dom[num][2][0],
&rpt_ptp_mtc.ptp_dom[num][3][0]);
send_ok = send(client, sendbuf, strlen(sendbuf), MSG_NOSIGNAL);
if(send_ok == -1 && errno == EAGAIN)
{
printf("timeout_<3>!\n");
}
return 0;
}
void set_sta_ip(unsigned const char *ip, unsigned char solt)
{
unsigned char r_ip[50];
unsigned char ip_temp[9];
unsigned char sendbuf[50];
if(NULL == ip)
{
return;
}
else
{
memset(sendbuf, 0, 50);
memset(r_ip, 0, 50);
memset(ip_temp, 0, 9);
memcpy(ip_temp, ip, 8);
sprintf((char *)sendbuf, "SET-STA1:000000::Board61850IP=");
}
#if 1
if(!read_ip(ip_temp, r_ip))
{
memset(conf_content3.sta1.ip, 0, 9);
memcpy(conf_content3.sta1.ip, ip_temp, 8);
sprintf((char *)sendbuf, "%s%s;", sendbuf, r_ip);
sendtodown(sendbuf, solt);
}
else
{
}
#endif
}
void set_sta_gateway(unsigned char *ip, unsigned char solt)
{
unsigned char r_ip[50];
unsigned char sendbuf[SENDBUFSIZE];
if(NULL == ip)
{
return;
}
else
{
memset(sendbuf, 0, SENDBUFSIZE);
memset(r_ip, 0, 50);
sprintf((char *)sendbuf, "SET-STA1:000000::Board61850GateWay=");
}
if(!read_ip(ip, r_ip))
{
memset(conf_content3.sta1.gate, 0, 9);
memcpy(conf_content3.sta1.gate, ip, 8);
sprintf((char *)sendbuf, "%s%s;", sendbuf, r_ip);
sendtodown(sendbuf, solt);
}
else
{
//;
}
}
void set_sta_netmask(unsigned char *ip, unsigned char solt)
{
unsigned char r_ip[50];
unsigned char sendbuf[SENDBUFSIZE];
if(NULL == ip)
{
return;
}
else
{
memset(sendbuf, 0, SENDBUFSIZE);
memset(r_ip, 0, 50);
sprintf((char *)sendbuf, "SET-STA1:000000::Board61850NetMask=");
}
if(!read_ip(ip, r_ip))
{
memset(conf_content3.sta1.mask, 0, 9);
memcpy(conf_content3.sta1.mask, ip, 8);
sprintf((char *)sendbuf, "%s%s;", sendbuf, r_ip);
sendtodown(sendbuf, solt);
}
else
{
//;
}
}
/*
SET-STA1-NET:
[<tid>]:<aid>:<ctag>:<password>:
<type>,<num>,<ip>,<mac>,<gateway>,<netmask>,<dns1>,<dns2>;
*/
int cmd_set_sta_net(_MSG_NODE *msg, int flag)
{
unsigned char *p = NULL;
unsigned char comma_id = 0;
signed char len;
printf("cmd_set_sta_net data:\n%s\n", msg->data);
printf("slot:%c\n", msg->solt);
if( ((msg->solt) < 'a') || ((msg->solt) > 'm') )
{
return 1;
}
else
{
p = msg->data;
len = strlen(msg->data);
}
while( (len>=0) && ((*p) != ';'))
{
len --;
if(',' == (*p))
{
comma_id++;
switch (comma_id)
{
case 0 :
//tpye
break;
case 1 :
//num
break;
case 2 ://ip
if( *(p+1) != ',' && *(p+1) != ';' )
{
set_sta_ip((p+1), msg->solt);
}
break;
case 3 ://mac
break;
case 4 ://gateway
if( *(p + 1) != ',' && *(p + 1) != ';' )
{
set_sta_gateway((p + 1), msg->solt);
}
break;
case 5 ://mask
if( *(p + 1) != ',' && *(p + 1) != ';' )
{
set_sta_netmask((p + 1), msg->solt);
}
break;
default://others
break;
}
}
else
{
;
}
p++;
}
if(flag)
{
//sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
//sendtodown(sendbuf, msg->solt);
}
respond_success(current_client, msg->ctag);
return 0;
}
//
//!set pgein net parameters
//
int cmd_set_pgein_net(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num;
int port;
int i, j, i1, i2, i3;
int len = strlen(msg->data);
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
memset(sendbuf, '\0', SENDBUFSIZE);
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
if((msg->data[2] == '1') )
{
num = (int)(msg->solt - 0x61);
port = (int)(msg->data[2] - 0x31);
for(i = 0; i < len; i++)
{
if(msg->data[i] == ',')
{
j++;
if(j == 2)
{
i1 = i + 1;
}
if(j == 3)
{
i2 = i + 1;
}
if(j == 4)
{
i3 = i + 1;
}
}
}
if(i3 == 0)
{
return 1;
}
conf_content.slot[num].ptp_type = msg->data[0];
if(msg->data[i1] != ',')
{
memcpy(conf_content.slot[num].ptp_ip[port], &msg->data[i1], i2 - i1 - 1);
conf_content.slot[num].ptp_ip[port][8] = '\0';
}
if(msg->data[i2] != ',')
{
memcpy(conf_content.slot[num].ptp_gate[port], &msg->data[i2], i3 - i2 - 1);
conf_content.slot[num].ptp_gate[port][8] = '\0';
}
if(msg->data[i3] != '\0')
{
memcpy(conf_content.slot[num].ptp_mask[port], &msg->data[i3], len - i3);
conf_content.slot[num].ptp_mask[port][8] = '\0';
}
sprintf((char *)sendbuf, "SET-PGEIN-NET:%s;", msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
// }
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
//
//!set pgein mode parameters
//
int cmd_set_pgein_mod(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num;
int port;
int i, j, i1, i2, i3, i4, i5, i6;
int len = strlen(msg->data);
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
i5 = 0;
i6 = 0;
memset(sendbuf, '\0', SENDBUFSIZE);
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
if((msg->data[2] == '1') )
{
num = (int)(msg->solt - 0x61);
port = (int)(msg->data[2] - 0x31);
for(i = 0; i < len; i++)
{
if(msg->data[i] == ',')
{
j++;
if(j == 2)
{
i1 = i + 1;
}
if(j == 3)
{
i2 = i + 1;
}
if(j == 4)
{
i3 = i + 1;
}
if(j == 5)
{
i4 = i + 1;
}
if(j == 6)
{
i5 = i + 1;
}
if(j == 7)
{
i6 = i + 1;
}
}
}
if(i6 == 0)
{
return 1;
}
conf_content.slot[num].ptp_type = msg->data[0];
//
//! set delaytype: 0-P2P, 1-E2E;
//
if((msg->data[i1] == '0') || (msg->data[i1] == '1'))
{
conf_content.slot[num].ptp_delaytype[port] = msg->data[i1];
}
//
//! 0-unicast,1-multicast;
//
if((msg->data[i2] == '0') || (msg->data[i2] == '1'))
{
conf_content.slot[num].ptp_multicast[port] = msg->data[i2];
}
//
//! enq : 0-two,1-three
//
if((msg->data[i3] == '0') || (msg->data[i3] == '1'))
{
conf_content.slot[num].ptp_enp[port] = msg->data[i3];
}
//
//!0:one step,1:two step;
//
if((msg->data[i4] == '0') || (msg->data[i4] == '1'))
{
conf_content.slot[num].ptp_step[port] = msg->data[i4];
}
//
//!delay req fre
//
if(msg->data[i5] != ',')
{
memcpy(conf_content.slot[num].ptp_delayreq[port], &msg->data[i5], i6 - i5 - 1);
conf_content.slot[num].ptp_delayreq[port][2] = '\0';
}
//
//!pdelay req fre
//
if(msg->data[i6] != '\0')
{
memcpy(conf_content.slot[num].ptp_pdelayreq[port], &msg->data[i6], len - i6);
conf_content.slot[num].ptp_pdelayreq[port][2] = '\0';
}
//
//!SET PGEIN MOD
//
sprintf((char *)sendbuf, "SET-PGEIN-MOD:%s;", msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
// }
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("PI MOD %s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
//
//!set pge4s net parameters
//
int cmd_set_pge4v_net(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
unsigned char datalen;
unsigned char errflag;
int num;
int port;
int i, j, i1, i2, i3, i4;
int len = strlen(msg->data);
datalen = 0;
errflag = 0;
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
//
//!Clear the snedbuf as null.
memset(sendbuf, '\0', SENDBUFSIZE);
//
//!the slot num le 14 and ge 1.
if(((msg->solt) > '`') && ((msg->solt) <= 'o'))
{
//
//check pge port le MAX_PORT_NUM.
//
if((msg->data[2] > '0') && (msg->data[2] <= MAX_PORT_NUM))
{
//
//Calculate the slot num and port num.
//
num = (int)(msg->solt - 0x61);
port = (int)(msg->data[2] - 0x31);
//
//! find the conntect (mac、IP、netmask)
//
for(i = 0; i < len; i++)
{
if(msg->data[i] == ',')
{
j++;
if(j == 2)
{
i1 = i + 1;
}
if(j == 3)
{
i2 = i + 1;
}
if(j == 4)
{
i3 = i + 1;
}
if(j == 5)
{
i4 = i + 1;
}
}
}
//
//if i4 equal to 0,the data frame is null。
//
if(i4 == 0)
{
return 1;
}
//
//save the ptp type of slot.
//
conf_content.slot[num].ptp_type = msg->data[0];
//
//Judge the data len or not correct.
//
datalen = i2 - i1 -1;
if(datalen > MAX_MAC_LEN)
errflag = 1;
datalen = i3 - i2 -1;
if(datalen > MAX_IP_LEN)
errflag = 1;
datalen = i4 - i3 -1;
if(datalen > MAX_IP_LEN)
errflag = 1;
datalen = len - i4;
if(datalen > MAX_IP_LEN)
errflag = 1;
if(1 == errflag)
return 1;
//
//save the mac parameters.
//
if(msg->data[i1] != ',')
{
memset(conf_content.slot[num].ptp_mac[port],'\0',18);
memcpy(conf_content.slot[num].ptp_mac[port], &msg->data[i1], i2 - i1 - 1);
}
//
//save the ip address.
//
if(msg->data[i2] != ',')
{
memset(conf_content.slot[num].ptp_ip[port],'\0',16);
memcpy(conf_content.slot[num].ptp_ip[port], &msg->data[i2], i3 - i2 - 1);
}
//
//save the mask address.
//
if(msg->data[i3] != ',')
{
memset(conf_content.slot[num].ptp_mask[port],'\0',16);
memcpy(conf_content.slot[num].ptp_mask[port], &msg->data[i3], i4 - i3 - 1);
}
//
//save the gate address.
//
if(msg->data[i4] != '\0')
{
memset(conf_content.slot[num].ptp_gate[port],'\0',16);
memcpy(conf_content.slot[num].ptp_gate[port], &msg->data[i4], len - i4);
}
sprintf((char *)sendbuf, "SET-PGE4V-NET:%s;", msg->data);
printf("SET-PGE4V-NET %s\n",msg->data);
//
// sned down to uart.
//
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
//
//! set the pge4s work mode.
//
int cmd_set_pge4v_mod(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num;
int port;
int i, j, i1, i2, i3, i4, i5, i6; /* i7, i8, i9, i10, i11, i12, i13;*/
int len = strlen(msg->data);
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
i5 = 0;
i6 = 0;
/*i7 = 0;
i8 = 0;
i9 = 0;
i10 = 0;
i11 = 0;
i12 = 0;
i13 = 0;*/
//
//clear sendbuf .
//
memset(sendbuf, '\0', SENDBUFSIZE);
//
//judge slot num is le 14 .
//
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
//
//judge the port num is le MAX_PORT_NUM。
//
if((msg->data[2] > '0') && (msg->data[2] <= MAX_PORT_NUM))
{
//
//!Calculate the num or port.
//
num = (int)(msg->solt - 0x61);
port = (int)(msg->data[2] - 0x31);
/*SET-PGE4S-MOD:<type>,<response message>;*/
//
//!
//
for(i = 0; i < len; i++)
{
if(msg->data[i] == ',')
{
j++;
if(j == 2)
{
i1 = i + 1;
}
if(j == 3)
{
i2 = i + 1;
}
if(j == 4)
{
i3 = i + 1;
}
if(j == 5)
{
i4 = i + 1;
}
if(j == 6)
{
i5 = i + 1;
}
if(j == 7)
{
i6 = i + 1;
}
}
}
if(i5 == 0)
{
return 1;
}
conf_content.slot[num].ptp_type = msg->data[0];
//
//!save the ptp enable.
//
if((msg->data[i1] == '0') || (msg->data[i1] == '1'))
{
conf_content.slot[num].ptp_en[port] = msg->data[i1];
}
//
//!save the esmc enable.
//
if((msg->data[i2] == '0') || (msg->data[i2] == '1'))
{
conf_content3.ptp_esmcen[num][port] = msg->data[i2];
//conf_content.slot[num].ptp_delaytype[port] = msg->data[i2];
}
//
//!save the delay type.
//
if((msg->data[i3] == '0') || (msg->data[i3] == '1'))
{
conf_content.slot[num].ptp_delaytype[port] = msg->data[i3];
}
//
//!save the multicast/unicast.
//
if((msg->data[i4] == '0') || (msg->data[i4] == '1'))
{
conf_content.slot[num].ptp_multicast[port] = msg->data[i4];
}
//
//!save the enq .
//
if((msg->data[i5] == '0') || (msg->data[i5] == '1'))
{
conf_content.slot[num].ptp_enp[port] = msg->data[i5];
}
//
//!save the step.
//
if((msg->data[i6] == '0') || (msg->data[i6] == '1'))
{
conf_content.slot[num].ptp_step[port] = msg->data[i5];
}
//
//!send the command down to uart.
//
sprintf((char *)sendbuf, "SET-PGE4V-MOD:%s;", msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
// }
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
//
//!set pge4s work paramters(Extern)。
//
int cmd_set_pge4v_par(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num;
int port;
int i, j, i1, i2, i3, i4, i5;/*, i6, i7, i8, i9, i10, i11, i12, i13;*/
int len = strlen(msg->data);
unsigned char datalen = 0;
unsigned errflag = 0;
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
i5 = 0;
/*i6 = 0;
i7 = 0;
i8 = 0;
i9 = 0;
i10 = 0;
i11 = 0;
i12 = 0;
i13 = 0;*/
//
//!clear send buffer.
//
memset(sendbuf, '\0', SENDBUFSIZE);
//
//judge the slot le 14 。
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
//
//limit the port range 1 ~ 4
if((msg->data[2] > '0') && (msg->data[2] <= MAX_PORT_NUM))
{
//
//Calculate slot number and Port number。
num = (int)(msg->solt - 0x61);
port = (int)(msg->data[2] - 0x31);
//
//Calculate the paramters position。
for(i = 0; i < len; i++)
{
if(msg->data[i] == ',')
{
j++;
if(j == 2)
{
i1 = i + 1;
}
if(j == 3)
{
i2 = i + 1;
}
if(j == 4)
{
i3 = i + 1;
}
if(j == 5)
{
i4 = i + 1;
}
if(j == 6)
{
i5 = i + 1;
}
}
}
if(i5 == 0)
{
return 1;
}
//
//judge the domain len is or not gt 3.
//
datalen = i2 - i1 -1;
if(datalen > MAX_DOM_LEN)
errflag = 1;
//
//judge the sync frequency len or not eq 2/0。
//
datalen = i3 - i2 -1;
if((datalen != FRAME_FRE_LEN)&&(datalen != 0))
errflag = 1;
//
//judge the announce frequency len or not eq 2/0。
//
datalen = i4 - i3 -1;
if((datalen != FRAME_FRE_LEN)&&(datalen != 0))
errflag = 1;
//
//judge the delaycon gt 7。
//
datalen = i5 - i4 -1;
if((datalen > DELAY_COM_LEN))
errflag = 1;
//
//judge the prio gt 4
datalen = len -i5;
if((datalen > MAX_PRIO_LEN))
errflag = 1;
if(1 == errflag)
return 1;
//
//slot type。
//
conf_content.slot[num].ptp_type = msg->data[0];
//
//save the domain number。
//
if(msg->data[i1] != ',')
{
memset(conf_content3.ptp_dom[num][port], 0, 4);
memcpy(conf_content3.ptp_dom[num][port], &msg->data[i1], i2 - i1 -1);
}
//
//save the sync frequency 。
//
if(msg->data[i2] != ',')
{
memcpy(conf_content.slot[num].ptp_sync[port], &msg->data[i2], i3 - i2 - 1);
}
//
//save the annouce frequency。
//
if(msg->data[i3] != ',')
{
memcpy(conf_content.slot[num].ptp_announce[port], &msg->data[i3], i4 - i3 - 1);
}
//
//save the delaycom。
//
if(msg->data[i4] != ',')
{
memset(conf_content.slot[num].ptp_delaycom[port],'\0',9);
memcpy(conf_content.slot[num].ptp_delaycom[port], &msg->data[i4], i5 - i4 - 1);
}
//
//save the pro。
//
if(msg->data[i5] != '\0')
{
if(msg->data[i5] == 'A')
{
memset(conf_content3.ptp_prio1[num][port], 0, 5);
memcpy(conf_content3.ptp_prio1[num][port], &msg->data[i5], len - i5);
}
else if(msg->data[i5] == 'B')
{
memset(conf_content3.ptp_prio2[num][port], 0, 5);
memcpy(conf_content3.ptp_prio2[num][port], &msg->data[i5], len - i5);
}
else
{
return 1;
}
}
sprintf((char *)sendbuf, "SET-PGE4V-PAR:%s;", msg->data);
printf("SET-PGE4V-PAR %s\n",msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
//
//! set pge4s mtc.
//
int cmd_set_pge4v_mtc(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num;
int port;
memset(sendbuf, '\0', SENDBUFSIZE);
//
//slot num.
//
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
if((msg->data[2] > '0') && (msg->data[2] <= MAX_PORT_NUM))
{
num = (int)(msg->solt - 0x61);
port = (int)(msg->data[2] - 0x31);
if(msg->data[4] != '\0')
{
memset(conf_content3.ptp_mtc_ip[num][port], 0, 9);
memcpy(conf_content3.ptp_mtc_ip[num][port], &msg->data[4], MAX_IP_LEN);
sprintf((char *)sendbuf, "SET-PGE4V-MTC:%s;", msg->data);
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
return 0;
}
return 1;
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
}
//
//!set pge4s work sfp modual(Extern)。
//
int cmd_set_pge4v_sfp(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
int num;
int port;
int i, j, i1, i2, i3;/*, i4, i5, i6, i7, i8, i9, i10, i11, i12, i13;*/
int len = strlen(msg->data);
unsigned char datalen = 0;
unsigned errflag = 0;
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
//
//!clear send buffer.
//
memset(sendbuf, '\0', SENDBUFSIZE);
//
//judge the slot le 14 。
if(((msg->solt) > '`') && ((msg->solt) < 'o'))
{
//
//limit the port range 1 ~ 4
if((msg->data[2] > '0') && (msg->data[2] <= MAX_PORT_NUM))
{
//
//Calculate slot number and Port number。
num = (int)(msg->solt - 0x61);
port = (int)(msg->data[2] - 0x31);
//
//Calculate the paramters position。
for(i = 0; i < len; i++)
{
if(msg->data[i] == ',')
{
j++;
if(j == 2)
{
i1 = i + 1;
}
if(j == 3)
{
i2 = i + 1;
}
if(j == 4)
{
i3 = i + 1;
}
}
}
if(i3 == 0)
{
return 1;
}
//
//judge the sfp modual setting len is or not gt 3.
//
datalen = i2 - i1 -1;
if((datalen != 2)&&(datalen != 0))
errflag = 1;
if(1 == errflag)
return 1;
//
//slot type。
//
conf_content.slot[num].ptp_type = msg->data[0];
//
//save the domain number。
//
if(msg->data[i1] != ',')
{
memset(conf_content3.ptp_sfp[num][port], 0, 3);
memcpy(conf_content3.ptp_sfp[num][port], &msg->data[i1], i2 - i1 -1);
}
//
//save the sfp modul op low thresh 。
//
if(msg->data[i2] != ',')
{
memset(conf_content3.ptp_oplo[num][port], 0, 7);
memcpy(conf_content3.ptp_oplo[num][port], &msg->data[i2], i3 - i2 - 1);
}
//
//save the sfp modul op hi thresh 。
//
if(msg->data[i3] != '\0')
{
memset(conf_content3.ptp_ophi[num][port], 0, 7);
memcpy(conf_content3.ptp_ophi[num][port], &msg->data[i2], len - i3);
}
//
//send down to uart 。
sprintf((char *)sendbuf, "SET-PGE4V-SFP:%s;", msg->data);
printf("SET-PGE4V-SFP %s\n",msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
}
}
else
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
/*
*********************************************************************************************************
* Function: int cmd_set_gb_mode(_MSG_NODE *msg, int flag)
* Descript: gbtpv2 work mode setting.
* Parameters: - msg :the point of input data.
* - flag :1:NMG SETTING; 0:Init Setting.
* Return: - 0 : ok
* - 1 : error
*
*********************************************************************************************************
*/
int cmd_set_gb_mode(_MSG_NODE *msg, int flag)
{
int len;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
len = strlen(msg->data);
if((strncmp1(msg->data,"GPS",3) != 0)&&(strncmp1(msg->data,"BD",3) != 0)&&(strncmp1(msg->data,"MIX",3) != 0))
return 1;
if(msg->solt == GBTP1_SLOT)
{
memset(conf_content.slot_q.mode, '\0', 4);
memcpy(conf_content.slot_q.mode, msg->data, len);
}
else if(msg->solt == GBTP2_SLOT)
{
memset(conf_content.slot_r.mode, '\0', 4);
memcpy(conf_content.slot_r.mode, msg->data, len);
}
else
{
return 1;
}
sprintf((char *)sendbuf, "SET-GBTP-MODE:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
/*
*********************************************************************************************************
* Function: int cmd_set_gn_mode(_MSG_NODE *msg, int flag)
* Descript: gbtpv2 work mode setting.
* Parameters: - msg :the point of input data.
* - flag :1:NMG SETTING; 0:Init Setting.
* Return: - 0 : ok
* - 1 : error
*
*********************************************************************************************************
*/
int cmd_set_gn_mode(_MSG_NODE *msg, int flag)
{
int len;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
len = strlen(msg->data);
if((strncmp1(msg->data,"GPS",3) != 0)&&(strncmp1(msg->data,"BD",3) != 0)&&(strncmp1(msg->data,"MIX",3) != 0)
&&(strncmp1(msg->data,"GLO",3)!= 0)&&(strncmp1(msg->data,"GAL",3)!= 0))
return 1;
if(msg->solt == GBTP1_SLOT)
{
memset(conf_content.slot_q.mode, '\0', 4);
memcpy(conf_content.slot_q.mode, msg->data, len);
}
else if(msg->solt == GBTP2_SLOT)
{
memset(conf_content.slot_r.mode, '\0', 4);
memcpy(conf_content.slot_r.mode, msg->data, len);
}
else
{
return 1;
}
sprintf((char *)sendbuf, "SET-GBTP-MODE:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
/*
*********************************************************************************************************
* Function: int cmd_set_gb_en(_MSG_NODE *msg, int flag)
* Descript: gbtpv2 work mode setting.
* Parameters: - msg :the point of input data.
* - flag :1:NMG SETTING; 0:Init Setting.
* Return: - 0 : ok
* - 1 : error
*
*********************************************************************************************************
*/
int cmd_set_gb_en(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
if((msg->data[0] != '0') && (msg->data[0] != '1'))
{
return 1;
}
if(msg->solt == GBTP1_SLOT)
{
memset(conf_content.slot_q.mask, '\0', 3);
memcpy(conf_content.slot_q.mask, msg->data, 2);
}
else if(msg->solt == GBTP2_SLOT)
{
memset(conf_content.slot_r.mask, '\0', 3);
memcpy(conf_content.slot_r.mask, msg->data, 2);
}
else
{
return 1;
}
sprintf((char *)sendbuf, "SET-GBTP-SATEN:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
/*
*********************************************************************************************************
* Function: int cmd_set_gn_dly(_MSG_NODE *msg, int flag)
* Descript: gbtpv2 work mode setting.
* Parameters: - msg :the point of input data.
* - flag :1:NMG SETTING; 0:Init Setting.
* Return: - 0 : ok
* - 1 : error
*
*********************************************************************************************************
*/
int is_digit1(unsigned char *parg,unsigned char len)
{
int i;
int usError;
usError = 0;
for(i = 0; i < len; i++)
{
if(i == 0)
{
if(parg[0] != '-')
{
if(parg[0] < 0x30 || parg[0] > 0x39)
{
usError = 1;
break;
}
}
}
else if(parg[i] < 0x30 || parg[i] > 0x39)
{
usError = 1;
break;
}
}
return usError;
}
int cmd_set_gn_dly(_MSG_NODE *msg, int flag)
{
int len, tmp_value;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
len = strlen(msg->data);
/*set delay rang -32768 ~ 32767*/
if(len <= 0 || len > 6)
return 1;
/*judge the ascii (0 ~ 9)*/
if(is_digit1(msg->data, len))
return 1;
/*judge delay rang -32768 ~ 32767*/
tmp_value = atoi(msg->data);
if(tmp_value < -32768 || tmp_value > 32767)
return 1;
if(msg->solt == GBTP1_SLOT)
{
memcpy(conf_content3.gbtp_delay[0], msg->data, len);
conf_content3.gbtp_delay[0][len] = '\0';
}
else if(msg->solt == GBTP2_SLOT)
{
memcpy(conf_content3.gbtp_delay[1], msg->data, len);
conf_content3.gbtp_delay[1][len] = '\0';
}
else
{
return 1;
}
sprintf((char *)sendbuf, "SET-GBTP-DELAY:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
/*GBTPIII 1PPS OUT DELAY,RANGE OF -400000~ 400000,UNIT 2.5ns*/
int cmd_set_pps_dly(_MSG_NODE *msg, int flag)
{
int len, tmp_value;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
len = strlen(msg->data);
/*set delay rang -400000 ~ 400000*/
if(len <= 0 || len > 10)
return 1;
/*judge the ascii (0 ~ 9)*/
if(is_digit1(msg->data, len))
return 1;
/*judge delay rang -200,000,000 ~ 200,000,000*/
tmp_value = atoi(msg->data);
if(tmp_value < -200000000 || tmp_value > 200000000)
return 1;
if(msg->solt == GBTP1_SLOT)
{
memcpy(conf_content3.gbtp_pps_delay[0], msg->data, len);
conf_content3.gbtp_pps_delay[0][len] = '\0';
}
else if(msg->solt == GBTP2_SLOT)
{
memcpy(conf_content3.gbtp_pps_delay[1], msg->data, len);
conf_content3.gbtp_pps_delay[1][len] = '\0';
}
else
{
return 1;
}
sprintf((char *)sendbuf, "SET-PPS-DELAY:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s\n", NET_INFO, sendbuf);
}
#endif
return 0;
}
/*
2015-9-9
*/
int cmd_set_protocol(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
unsigned char i;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
if((msg->data[0] != '0') && (msg->data[0] != '1') && (msg->data[0] != '2'))
{
return 1;
}
else
{
conf_content3.mcp_protocol = msg->data[0];
}
printf("### cmd_set_protocol:%c\n",msg->data[0]);
sprintf((char *)sendbuf, "SET-PROTOCOL:%s::%c;", msg->ctag, msg->data[0]);
for(i=0;i<13;i++)
{
printf("### %d--type:%c\n",i,slot_type[i]);
if( 'I' == slot_type[i] || 'j' == slot_type[i] || //TP16 PGE4V2
(slot_type[i] >= 'A' && slot_type[i] <= 'H') || //PTP4\PFO4\PGE4\PGO4\PTP8\PFO8\PGE8\PGO8
(slot_type[i] >= 'n' && slot_type[i] <= 'q') ) //PTP\PGE\PTP2\PGE2
{
printf("### SENDTODOWN:%s\n",sendbuf);
if(flag)
{
sendtodown_cli(sendbuf, (char)('a'+i), msg->ctag);
}
else
{
sendtodown(sendbuf, (char)('a'+i));
}
}
else
{
continue;
}
}
save_config();
respond_success(current_client, msg->ctag);
return 0;
}
int cmd_set_ppssel(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
//unsigned char i;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
if((msg->data[0] < '0') || (msg->data[0] > '6'))
{
return 1;
}
if(msg->solt == RB1_SLOT)
{
conf_content3.pps_sel[0] = msg->data[0];
}
else if(msg->solt == RB2_SLOT)
{
conf_content3.pps_sel[1] = msg->data[0];
}
else
{
return 1;
}
sprintf((char *)sendbuf, "SET-PPS-SEL:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
respond_success(current_client, msg->ctag);
return 0;
}
int cmd_set_tdeven(_MSG_NODE *msg, int flag)
{
unsigned char sendbuf[SENDBUFSIZE];
//unsigned char i;
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
if((msg->data[0] !='0') && (msg->data[0] != '1'))
{
return 1;
}
if(msg->solt == RB1_SLOT)
{
conf_content3.tdev_en[0] = msg->data[0];
}
else if(msg->solt == RB2_SLOT)
{
conf_content3.tdev_en[1] = msg->data[0];
}
else
{
return 1;
}
sprintf((char *)sendbuf, "SET-TDEV-EN:%s::%s;", msg->ctag, msg->data);
if(flag)
{
sendtodown_cli(sendbuf, msg->solt, msg->ctag);
save_config();
}
else
{
sendtodown(sendbuf, msg->solt);
}
respond_success(current_client, msg->ctag);
return 0;
}
_CMD_NODE g_cmd_fun[] =
{
//扩展框
{"QEXT-1-EQT", 10, NULL, cmd_ext1_eqt},
{"QEXT-2-EQT", 10, NULL, cmd_ext2_eqt},
{"QEXT-3-EQT", 10, NULL, cmd_ext3_eqt},
{"QEXT-EQT",8,NULL,cmd_ext_eqt},
{"QEXT-1-ALM", 10, NULL, cmd_ext1_alm},
{"QEXT-2-ALM", 10, NULL, cmd_ext2_alm},
{"QEXT-3-ALM", 10, NULL, cmd_ext3_alm},
{"QEXT-ALM", 8, NULL, cmd_ext_alm},
{"QEXT-1-PRS", 10, NULL, cmd_ext1_prs},
{"QEXT-2-PRS", 10, NULL, cmd_ext2_prs},
{"QEXT-3-PRS", 10, NULL, cmd_ext3_prs},
{"QEXT-DRV-MGR", 12, NULL, cmd_drv_mgr},
{"QEXT-ONLINE", 11, NULL, cmd_qext_online},
{"SET-EXT-ONLINE", 14, cmd_set_ext_online, NULL},
{"RTRV-ALRMS", 10, NULL, cmd_rtrv_alrms},
{"RTRV-EQPT", 9, NULL, cmd_rtrv_eqpt},
{"RTRV-PM-INP", 11, NULL, cmd_rtrv_pm_inp},
{"RTRV-PM-MSG", 11, NULL, cmd_rtrv_pm_msg},
{"RTRV-OUT", 8, NULL, cmd_rtrv_out},
{"RTRV-MTC-IP", 11, NULL, cmd_rtrv_mtc_ip},
{"RTRV-UNI-IP", 11, NULL, cmd_rtrv_uni_ip},
{"RTRV-MTC-DOM", 12, NULL, cmd_rtrv_mtc_dom},
{"SET-TID", 7, cmd_set_tid, NULL},
{"HAND-SHAKE", 10, cmd_hand_shake, NULL},
{"SET-MAIN", 8, cmd_set_main, NULL},
{"SET-DAT_S", 9, cmd_set_dat_s, NULL},
{"SET-DAT", 7, cmd_set_dat, NULL},
{"SET-NET", 7, cmd_set_net, NULL},
{"SET-SSM-OEN", 11, cmd_set_out_ssm_en, NULL},
{"SET-SSM-OTH", 11, cmd_set_out_ssm_oth, NULL},
{"SET-MAC", 7, cmd_set_mac, NULL},
{"ED-USER-SECU", 12, cmd_set_password, NULL},
{"SET-MODE", 8, cmd_set_mode, NULL},
{"SET-POS", 7, cmd_set_pos, NULL},
{"SET-MASK-GBTP", 13, cmd_mask_gbtp, NULL},
{"SET-BDZB", 8, cmd_bdzb, NULL},
{"SET-SYS-REF", 11, cmd_sys_ref, NULL},
{"SET-DELY", 8, cmd_set_dely, NULL},
{"SET-PRIO-INP", 12, cmd_set_prio_inp, NULL},
{"SET-MASK-E1", 11, cmd_set_mask_e1, NULL},
{"SET-LEAP-MASK", 13, cmd_set_leap_mask, NULL},
{"SET-TZO", 7, cmd_set_tzo, NULL},
{"SET-PTP-NET", 11, cmd_set_ptp_net, NULL},
{"SET-PTP-MOD", 11, cmd_set_ptp_mod, NULL},
{"SET-PTP-MTC", 11, cmd_set_ptp_mtc, NULL},
{"SET-PTP-DOM",11, cmd_set_ptp_dom,NULL},//添加PTP时钟域号设置。
{"SET-OUT-LEV", 11, cmd_set_out_lev, NULL},
{"SET-OUT-TYP", 11, cmd_set_out_typ, NULL},
{"SET-OUT-PRR", 11, cmd_set_out_ppr, NULL},
{"SET-RESET", 9, cmd_set_reset, NULL},
{"SET-LEAP-TAG", 12, cmd_set_leap_tag, NULL},
{"SET-TP16-EN", 11, cmd_set_tp16_en, NULL},
{"SET-CONFIG", 10, cmd_set_CONFIG, NULL},
{"SET-LEAP-NUM", 12, cmd_set_leap_num, NULL},
{"SET-REF-PRIO", 12, cmd_set_ref_prio, NULL},
{"SET-REF-MOD", 11, cmd_set_ref_mod, NULL},
{"SET-REF-SA", 10, cmd_set_ref_sa, NULL},
{"SET-REF-PH", 10, cmd_set_ref_ph, NULL},
{"SET-REF-TLB", 11, cmd_set_ref_tlb, NULL},//增加2Mb信号等级设置,在2Mb设置为网管预设值时有效。
{"SET-REF-TLM", 11, cmd_set_ref_tlm, NULL},//增加2Mbit信号获取信号等级来源。
{"SET-REF-TL", 10, cmd_set_ref_tl, NULL},
{"SET-REF-IEN", 11, cmd_set_ref_ssm_en, NULL},
{"SET-RS-EN", 9, cmd_set_rs_en, NULL},
{"SET-RS-TZO", 10, cmd_set_rs_tzo, NULL},
{"SET-PPX-MOD", 11, cmd_set_ppx_mod, NULL},
{"SET-TOD-EN", 10, cmd_set_tod_en, NULL},
{"SET-TOD-PRO", 11, cmd_set_tod_pro, NULL},
{"SET-IGB-EN", 10, cmd_set_igb_en, NULL},
{"SET-IGB-RAT", 11, cmd_set_igb_rat, NULL},
{"SET-IGB-MAX", 11, cmd_set_igb_max, NULL},
//2015527 set IRIGR16 timezone
{"SET-IGB-TZONE", 13, cmd_set_igb_tzone, NULL},
{"SET-NTP-EN", 10, cmd_set_ntp_en, NULL},
{"SET-NTP-NET", 11, cmd_set_ntp_net, NULL},
{"SET-NTP-PNET", 12, cmd_set_ntp_pnet, NULL},//new add
{"SET-NTP-PMAC", 12, cmd_set_ntp_pmac, NULL},//2017/6/9 (new add)
{"SET-NTP-PEN", 11, cmd_set_ntp_pen, NULL}, //new add
{"SET-NTP-KEY", 11, cmd_set_ntp_key, NULL},
{"SET-NTP-MS", 10, cmd_set_ntp_ms, NULL},
{"SET-MCP-FB", 10, cmd_set_mcp_fb, NULL},
{"SET-RB-SA", 9, cmd_set_rb_sa, NULL},
{"SET-RB-TL", 9, cmd_set_rb_tl, NULL},
{"SET-RB-TH", 9, cmd_set_rb_thresh, NULL},//设置钟盘IRIGB设置门限
{"SET-OUT-MOD", 11, cmd_set_out_mod, NULL},
//设置告警屏蔽
{"SET-ARLM-MASK", 13, cmd_set_alm_msk, NULL},
{"RTRV-RALM-MASK", 14, NULL, cmd_rtrv_ralm_mask},
//20141210
{"SET-LEAP-MOD", 12, cmd_set_leap_mod, NULL},
{"RTRV-LEAP-MOD", 13, NULL, cmd_rtrv_leap_mod},
//20150527 查询irigb16时区
{"RTRV-IGB-TZONE", 13, NULL, cmd_rtrv_igb_tzone},
{"SYS-CPU", 7, NULL, cmd_sys_cpu},
//STA-1
{"SET-STA1-NET", 12, cmd_set_sta_net, NULL},
//PGEIN
{"SET-PGEIN-NET", 13, cmd_set_pgein_net, NULL},
{"SET-PGEIN-MOD", 13, cmd_set_pgein_mod, NULL},
//PGE4S SET-PGE4S-NET
{"SET-PGE4V-NET", 13, cmd_set_pge4v_net, NULL},
{"SET-PGE4V-MOD", 13, cmd_set_pge4v_mod, NULL},
{"SET-PGE4V-PAR", 13, cmd_set_pge4v_par, NULL},
{"SET-PGE4V-MTC", 13, cmd_set_pge4v_mtc, NULL},
{"SET-PGE4V-SFP", 13, cmd_set_pge4v_sfp, NULL},
//GBTPIIV2 SETTING
{"SET-GB-MODE", 11, cmd_set_gb_mode, NULL},
{"SET-GB-EN", 9, cmd_set_gb_en, NULL},
//GBTPIII SETTING
{"SET-GN-MODE", 11, cmd_set_gn_mode, NULL},
{"SET-GN-EN", 9, cmd_set_gb_en, NULL},
{"SET-GN-DLY", 10, cmd_set_gn_dly, NULL},
{"SET-PPS-DLY", 11, cmd_set_pps_dly, NULL},
//2015-9-9 联通移动版本选择
{"SET-PROTOCOL", 12, cmd_set_protocol, NULL},
{"SET-PPS-SEL", 11, cmd_set_ppssel, NULL},
{"SET-TDEV-EN", 11, cmd_set_tdeven, NULL},
};
int _CMD_NODE_MAX = ((int)(sizeof(g_cmd_fun) / sizeof(_CMD_NODE))); //2014.12.5
<file_sep>/MCPU/ext_trpt.c
#include <stdio.h>
#include <string.h>
#include "ext_ctx.h"
#include "ext_trpt.h"
#include "ext_cmn.h"
#include "mcp_process.h"
//#include "memwatch.h"
/*
1 成功
0 失败
*/
u8_t ext_out_ssm_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx)
{
int i, j;
int slotid;
u8_t sendbuf[128];
//printf("--%s--\n", ext_data);
if((EXT_1_MGR_PRI == ext_sid) || (EXT_1_MGR_RSV == ext_sid))
{
for(i = 0, j = 0; (i < 20) && (j < 10); i += 2, j++)
{
if( EXT_OUT16 == ctx->extBid[0][j] ||
EXT_OUT32 == ctx->extBid[0][j] ||
EXT_OUT16S == ctx->extBid[0][j] ||
EXT_OUT32S == ctx->extBid[0][j] )
{
if(' ' != ext_data[i] && ' ' != ext_data[i + 1])
{
memcpy(ctx->out[j].outSsm, &ext_data[i], 2);
//memcpy(ctx->save.outSsm[j], &ext_data[i], 2);
if(0 != memcmp(ctx->out[j].outSsm, ctx->save.outSsm[j], 2))
{
//lev
if(2 == strlen(ctx->save.outSsm[j]))
{
memcpy(ctx->out[j].outSsm, ctx->save.outSsm[j], 2);
memset(sendbuf, 0, 128);
sprintf((char *)sendbuf, "SET-OUT-LEV:%c:%s::%s;",
j + EXT_1_OUT_LOWER,
"000000",
ctx->save.outSsm[j]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, j + EXT_1_OUT_LOWER, "000000");
}
}
}
}
}
}
else if((EXT_2_MGR_PRI == ext_sid) || (EXT_2_MGR_RSV == ext_sid))
{
for(i = 0, j = 0; (i < 20) && (j < 10); i += 2, j++)
{
if( EXT_OUT16 == ctx->extBid[1][j] ||
EXT_OUT32 == ctx->extBid[1][j] ||
EXT_OUT16S == ctx->extBid[1][j] ||
EXT_OUT32S == ctx->extBid[1][j] )
{
if(' ' != ext_data[i] && ' ' != ext_data[i + 1])
{
memcpy(ctx->out[10 + j].outSsm, &ext_data[i], 2);
//memcpy(ctx->save.outSsm[10+j], &ext_data[i], 2);
if(0 != memcmp(ctx->out[10 + j].outSsm, ctx->save.outSsm[10 + j], 2))
{
//lev
if(2 == strlen(ctx->save.outSsm[10 + j]))
{
memcpy(ctx->out[10 + j].outSsm, ctx->save.outSsm[10 + j], 2);
memset(sendbuf, 0, 128);
sprintf((char *)sendbuf, "SET-OUT-LEV:%c:%s::%s;",
j + EXT_2_OUT_LOWER,
"000000",
ctx->save.outSsm[10 + j]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, j + EXT_2_OUT_LOWER, "000000");
}
}
}
}
}
}
else if((EXT_3_MGR_PRI == ext_sid) || (EXT_3_MGR_RSV == ext_sid))
{
for(i = 0, j = 0; (i < 20) && (j < 10); i += 2, j++)
{
if( EXT_OUT16 == ctx->extBid[2][j] ||
EXT_OUT32 == ctx->extBid[2][j] ||
EXT_OUT16S == ctx->extBid[2][j] ||
EXT_OUT32S == ctx->extBid[2][j] )
{
if(' ' != ext_data[i] && ' ' != ext_data[i + 1])
{
memcpy(ctx->out[20 + j].outSsm, &ext_data[i], 2);
//memcpy(ctx->save.outSsm[20+j], &ext_data[i], 2);
if(0 != memcmp(ctx->out[20 + j].outSsm, ctx->save.outSsm[20 + j], 2))
{
if((j + EXT_3_OUT_LOWER) >= ':')//except ':;'
{
slotid = j + EXT_3_OUT_LOWER + 2;
}
else
{
slotid = j + EXT_3_OUT_LOWER;
}
//lev
if(2 == strlen(ctx->save.outSsm[20 + j]))
{
memcpy(ctx->out[20 + j].outSsm, ctx->save.outSsm[20 + j], 2);
memset(sendbuf, 0, 128);
sprintf((char *)sendbuf, "SET-OUT-LEV:%c:%s::%s;",
slotid,
"000000",
ctx->save.outSsm[20 + j]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, slotid, "000000");
}
}
}
}
}
}
else
{
return 0;
}
return 1;
}
/*
1 成功
0 失败
*/
u8_t ext_out_signal_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx)
{
int i;
u8_t bid;
int slotid;
u8_t sendbuf[128];
/*
对于OUT16,分为两组(1-8 9-16),
bit0-bit3表示1-8路,bit4-bit7表示9-16路。
对于OUT32,分为四组(1-4 5-8 9-12 13-16),
bit0-bit1表示1-4路,bit2-bit3表示5-8路,bit4-bit5表示9-12路,bit6-bit7表示13-16路。
0:2.048Mhz
1:2.048Mbit/s
2:无输出
*/
if((EXT_1_MGR_PRI == ext_sid) || (EXT_1_MGR_RSV == ext_sid))
{
for(i = 0; i < 10; i++)
{
bid = ctx->extBid[0][i];
if(EXT_OUT16 == bid || EXT_OUT16S == bid)
{
if(0 == (ext_data[i] & 0x0F))
{
memcpy(ctx->out[i].outSignal, "00000000", 8);
}
else if(1 == (ext_data[i] & 0x0F))
{
memcpy(ctx->out[i].outSignal, "11111111", 8);
}
else if(2 == (ext_data[i] & 0x0F))
{
memcpy(ctx->out[i].outSignal, "22222222", 8);
}
else
{
return 0;
}
if(0 == ((ext_data[i] >> 4) & 0x0F))
{
memcpy(&(ctx->out[i].outSignal[8]), "00000000", 8);
}
else if(1 == ((ext_data[i] >> 4) & 0x0F))
{
memcpy(&(ctx->out[i].outSignal[8]), "11111111", 8);
}
else if(2 == ((ext_data[i] >> 4) & 0x0F))
{
memcpy(&(ctx->out[i].outSignal[8]), "22222222", 8);
}
else
{
return 0;
}
//memcpy(ctx->save.outSignal[i], ctx->out[i].outSignal, 16);
if(0 != memcmp(ctx->out[i].outSignal, ctx->save.outSignal[i], 16))
{
//signal
if(16 == strlen(ctx->save.outSignal[i]))
{
memcpy(ctx->out[i].outSignal, ctx->save.outSignal[i], 16);
memset(sendbuf, 0, 128);
sprintf((char *)sendbuf, "SET-OUT-TYP:%c:%s::%s;",
i + EXT_1_OUT_LOWER,
"000000",
ctx->save.outSignal[i]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, i + EXT_1_OUT_LOWER, "000000");
}
}
}
else if(EXT_OUT32 == bid || EXT_OUT32S == bid)
{
if(0 == (ext_data[i] & 0x03))
{
memcpy(ctx->out[i].outSignal, "0000", 4);
}
else if(1 == (ext_data[i] & 0x03))
{
memcpy(ctx->out[i].outSignal, "1111", 4);
}
else if(2 == (ext_data[i] & 0x03))
{
memcpy(ctx->out[i].outSignal, "2222", 4);
}
else
{
return 0;
}
if(0 == ((ext_data[i] >> 2) & 0x03))
{
memcpy(&(ctx->out[i].outSignal[4]), "0000", 4);
}
else if(1 == ((ext_data[i] >> 2) & 0x03))
{
memcpy(&(ctx->out[i].outSignal[4]), "1111", 4);
}
else if(2 == ((ext_data[i] >> 2) & 0x03))
{
memcpy(&(ctx->out[i].outSignal[4]), "2222", 4);
}
else
{
return 0;
}
if(0 == ((ext_data[i] >> 4) & 0x03))
{
memcpy(&(ctx->out[i].outSignal[8]), "0000", 4);
}
else if(1 == ((ext_data[i] >> 4) & 0x03))
{
memcpy(&(ctx->out[i].outSignal[8]), "1111", 4);
}
else if(2 == ((ext_data[i] >> 4) & 0x03))
{
memcpy(&(ctx->out[i].outSignal[8]), "2222", 4);
}
else
{
return 0;
}
if(0 == ((ext_data[i] >> 6) & 0x03))
{
memcpy(&(ctx->out[i].outSignal[12]), "0000", 4);
}
else if(1 == ((ext_data[i] >> 6) & 0x03))
{
memcpy(&(ctx->out[i].outSignal[12]), "1111", 4);
}
else if(2 == ((ext_data[i] >> 6) & 0x03))
{
memcpy(&(ctx->out[i].outSignal[12]), "2222", 4);
}
else
{
return 0;
}
//memcpy(ctx->save.outSignal[i], ctx->out[i].outSignal, 16);
if(0 != memcmp(ctx->out[i].outSignal, ctx->save.outSignal[i], 16))
{
//signal
if(16 == strlen(ctx->save.outSignal[i]))
{
memcpy(ctx->out[i].outSignal, ctx->save.outSignal[i], 16);
memset(sendbuf, 0, 128);
sprintf((char *)sendbuf, "SET-OUT-TYP:%c:%s::%s;",
i + EXT_1_OUT_LOWER,
"000000",
ctx->save.outSignal[i]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, i + EXT_1_OUT_LOWER, "000000");
}
}
}
else
{
//
}
}
}
else if((EXT_2_MGR_PRI == ext_sid) || (EXT_2_MGR_RSV == ext_sid))
{
for(i = 0; i < 10; i++)
{
bid = ctx->extBid[1][i];
if(EXT_OUT16 == bid || EXT_OUT16S == bid)
{
if(0 == (ext_data[i] & 0x0F))
{
memcpy(ctx->out[10 + i].outSignal, "00000000", 8);
}
else if(1 == (ext_data[i] & 0x0F))
{
memcpy(ctx->out[10 + i].outSignal, "11111111", 8);
}
else if(2 == (ext_data[i] & 0x0F))
{
memcpy(ctx->out[10 + i].outSignal, "22222222", 8);
}
else
{
return 0;
}
if(0 == ((ext_data[i] >> 4) & 0x0F))
{
memcpy(&(ctx->out[10 + i].outSignal[8]), "00000000", 8);
}
else if(1 == ((ext_data[i] >> 4) & 0x0F))
{
memcpy(&(ctx->out[10 + i].outSignal[8]), "11111111", 8);
}
else if(2 == ((ext_data[i] >> 4) & 0x0F))
{
memcpy(&(ctx->out[10 + i].outSignal[8]), "22222222", 8);
}
else
{
return 0;
}
//memcpy(ctx->save.outSignal[10+i], ctx->out[10+i].outSignal, 16);
if(0 != memcmp(ctx->out[10 + i].outSignal, ctx->save.outSignal[10 + i], 16))
{
//signal
if(16 == strlen(ctx->save.outSignal[10 + i]))
{
memcpy(ctx->out[10 + i].outSignal, ctx->save.outSignal[10 + i], 16);
memset(sendbuf, 0, 128);
sprintf((char *)sendbuf, "SET-OUT-TYP:%c:%s::%s;",
i + EXT_2_OUT_LOWER,
"000000",
ctx->save.outSignal[10 + i]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, i + EXT_2_OUT_LOWER, "000000");
}
}
}
else if(EXT_OUT32 == bid || EXT_OUT32S == bid)
{
if(0 == (ext_data[i] & 0x03))
{
memcpy(ctx->out[10 + i].outSignal, "0000", 4);
}
else if(1 == (ext_data[i] & 0x03))
{
memcpy(ctx->out[10 + i].outSignal, "1111", 4);
}
else if(2 == (ext_data[i] & 0x03))
{
memcpy(ctx->out[10 + i].outSignal, "2222", 4);
}
else
{
return 0;
}
if(0 == ((ext_data[i] >> 2) & 0x03))
{
memcpy(&(ctx->out[10 + i].outSignal[4]), "0000", 4);
}
else if(1 == ((ext_data[i] >> 2) & 0x03))
{
memcpy(&(ctx->out[10 + i].outSignal[4]), "1111", 4);
}
else if(2 == ((ext_data[i] >> 2) & 0x03))
{
memcpy(&(ctx->out[10 + i].outSignal[4]), "2222", 4);
}
else
{
return 0;
}
if(0 == ((ext_data[i] >> 4) & 0x03))
{
memcpy(&(ctx->out[10 + i].outSignal[8]), "0000", 4);
}
else if(1 == ((ext_data[i] >> 4) & 0x03))
{
memcpy(&(ctx->out[10 + i].outSignal[8]), "1111", 4);
}
else if(2 == ((ext_data[i] >> 4) & 0x03))
{
memcpy(&(ctx->out[10 + i].outSignal[8]), "2222", 4);
}
else
{
return 0;
}
if(0 == ((ext_data[i] >> 6) & 0x03))
{
memcpy(&(ctx->out[10 + i].outSignal[12]), "0000", 4);
}
else if(1 == ((ext_data[i] >> 6) & 0x03))
{
memcpy(&(ctx->out[10 + i].outSignal[12]), "1111", 4);
}
else if(2 == ((ext_data[i] >> 6) & 0x03))
{
memcpy(&(ctx->out[10 + i].outSignal[12]), "2222", 4);
}
else
{
return 0;
}
//memcpy(ctx->save.outSignal[10+i], ctx->out[10+i].outSignal, 16);
if(0 != memcmp(ctx->out[10 + i].outSignal, ctx->save.outSignal[10 + i], 16))
{
//signal
printf("2+%d+%s\n", i + 1, ctx->save.outSignal[10 + i]);
if(16 == strlen(ctx->save.outSignal[10 + i]))
{
memcpy(ctx->out[10 + i].outSignal, ctx->save.outSignal[10 + i], 16);
memset(sendbuf, 0, 128);
sprintf((char *)sendbuf, "SET-OUT-TYP:%c:%s::%s;",
i + EXT_2_OUT_LOWER,
"000000",
ctx->save.outSignal[10 + i]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, i + EXT_2_OUT_LOWER, "000000");
}
}
}
else
{
//
}
}
}
else if((EXT_3_MGR_PRI == ext_sid) || (EXT_3_MGR_RSV == ext_sid))
{
for(i = 0; i < 10; i++)
{
bid = ctx->extBid[2][i];
if(EXT_OUT16 == bid || EXT_OUT16S == bid)
{
if(0 == (ext_data[i] & 0x0F))
{
memcpy(ctx->out[20 + i].outSignal, "00000000", 8);
}
else if(1 == (ext_data[i] & 0x0F))
{
memcpy(ctx->out[20 + i].outSignal, "11111111", 8);
}
else if(2 == (ext_data[i] & 0x0F))
{
memcpy(ctx->out[20 + i].outSignal, "22222222", 8);
}
else
{
return 0;
}
if(0 == ((ext_data[i] >> 4) & 0x0F))
{
memcpy(&(ctx->out[20 + i].outSignal[8]), "00000000", 8);
}
else if(1 == ((ext_data[i] >> 4) & 0x0F))
{
memcpy(&(ctx->out[20 + i].outSignal[8]), "11111111", 8);
}
else if(2 == ((ext_data[i] >> 4) & 0x0F))
{
memcpy(&(ctx->out[20 + i].outSignal[8]), "22222222", 8);
}
else
{
return 0;
}
//memcpy(ctx->save.outSignal[20+i], ctx->out[20+i].outSignal, 16);
if(0 != memcmp(ctx->out[20 + i].outSignal, ctx->save.outSignal[20 + i], 16))
{
if((i + EXT_3_OUT_LOWER) >= ':')//except ':;'
{
slotid = i + EXT_3_OUT_LOWER + 2;
}
else
{
slotid = i + EXT_3_OUT_LOWER;
}
//signal
if(16 == strlen(ctx->save.outSignal[20 + i]))
{
memcpy(ctx->out[20 + i].outSignal, ctx->save.outSignal[20 + i], 16);
printf("3+%d+%s\n", i + 1, ctx->save.outSignal[20 + i]);
memset(sendbuf, 0, 128);
sprintf((char *)sendbuf, "SET-OUT-TYP:%c:%s::%s;",
slotid,
"000000",
ctx->save.outSignal[20 + i]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, slotid, "000000");
}
}
}
else if(EXT_OUT32 == bid || EXT_OUT32S == bid)
{
if(0 == (ext_data[i] & 0x03))
{
memcpy(ctx->out[20 + i].outSignal, "0000", 4);
}
else if(1 == (ext_data[i] & 0x03))
{
memcpy(ctx->out[20 + i].outSignal, "1111", 4);
}
else if(2 == (ext_data[i] & 0x03))
{
memcpy(ctx->out[20 + i].outSignal, "2222", 4);
}
else
{
return 0;
}
if(0 == ((ext_data[i] >> 2) & 0x03))
{
memcpy(&(ctx->out[20 + i].outSignal[4]), "0000", 4);
}
else if(1 == ((ext_data[i] >> 2) & 0x03))
{
memcpy(&(ctx->out[20 + i].outSignal[4]), "1111", 4);
}
else if(2 == ((ext_data[i] >> 2) & 0x03))
{
memcpy(&(ctx->out[20 + i].outSignal[4]), "2222", 4);
}
else
{
return 0;
}
if(0 == ((ext_data[i] >> 4) & 0x03))
{
memcpy(&(ctx->out[20 + i].outSignal[8]), "0000", 4);
}
else if(1 == ((ext_data[i] >> 4) & 0x03))
{
memcpy(&(ctx->out[20 + i].outSignal[8]), "1111", 4);
}
else if(2 == ((ext_data[i] >> 4) & 0x03))
{
memcpy(&(ctx->out[20 + i].outSignal[8]), "2222", 4);
}
else
{
return 0;
}
if(0 == ((ext_data[i] >> 6) & 0x03))
{
memcpy(&(ctx->out[20 + i].outSignal[12]), "0000", 4);
}
else if(1 == ((ext_data[i] >> 6) & 0x03))
{
memcpy(&(ctx->out[20 + i].outSignal[12]), "1111", 4);
}
else if(2 == ((ext_data[i] >> 6) & 0x03))
{
memcpy(&(ctx->out[20 + i].outSignal[12]), "2222", 4);
}
else
{
return 0;
}
//memcpy(ctx->save.outSignal[20+i], ctx->out[20+i].outSignal, 16);
if(0 != memcmp(ctx->out[20 + i].outSignal, ctx->save.outSignal[20 + i], 16))
{
if((i + EXT_3_OUT_LOWER) >= ':')//except ':;'
{
slotid = i + EXT_3_OUT_LOWER + 2;
}
else
{
slotid = i + EXT_3_OUT_LOWER;
}
//signal
if(16 == strlen(ctx->save.outSignal[20 + i]))
{
memcpy(ctx->out[20 + i].outSignal, ctx->save.outSignal[20 + i], 16);
printf("3+%d+%s\n", i + 1, ctx->save.outSignal[20 + i]);
memset(sendbuf, 0, 128);
sprintf((char *)sendbuf, "SET-OUT-TYP:%c:%s::%s;",
slotid,
"000000",
ctx->save.outSignal[20 + i]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, slotid, "000000");
}
}
}
else
{
//
}
}
}
else
{
return 0;
}
return 1;
}
/*
1 成功
0 失败
*/
u8_t ext_out_mode_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx)
{
int i, j;
int slotid;
u8_t sendbuf[128];
if((EXT_1_MGR_PRI == ext_sid) || (EXT_1_MGR_RSV == ext_sid))
{
for(i = 0, j = 0; i < 20 && j < 10; i += 2, j++)
{
if( EXT_OUT16 == ctx->extBid[0][j] ||
EXT_OUT32 == ctx->extBid[0][j] ||
EXT_OUT16S == ctx->extBid[0][j] ||
EXT_OUT32S == ctx->extBid[0][j] )
{
if(' ' != ext_data[i] && ' ' != ext_data[i + 1])
{
ctx->out[j].outPR[0] = ext_data[i];
ctx->out[j].outPR[1] = ',';
ctx->out[j].outPR[2] = ext_data[i + 1];
}
/*ctx->save.outPR[j][0] = ext_data[i];
ctx->save.outPR[j][1] = ',';
ctx->save.outPR[j][2] = ext_data[i+1];*/
if(0 != memcmp(ctx->out[j].outPR, ctx->save.outPR[j], 3))
{
//mod
printf("1+%d+%s\n", j + 1, ctx->save.outPR[j]);
if('0' == ctx->save.outPR[j][0] || '1' == ctx->save.outPR[j][0])
{
memcpy(ctx->out[j].outPR, ctx->save.outPR[j], 1);
memset(sendbuf, 0, 128);
sprintf((char *)sendbuf, "SET-OUT-MOD:%c:%s::%c;",
j + EXT_1_OUT_LOWER,
"000000",
ctx->save.outPR[j][0]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, j + EXT_1_OUT_LOWER, "000000");
}
}
}
}
}
else if((EXT_2_MGR_PRI == ext_sid) || (EXT_2_MGR_RSV == ext_sid))
{
for(i = 0, j = 0; i < 20 && j < 10; i += 2, j++)
{
if( EXT_OUT16 == ctx->extBid[1][j] ||
EXT_OUT32 == ctx->extBid[1][j] ||
EXT_OUT16S == ctx->extBid[1][j] ||
EXT_OUT32S == ctx->extBid[1][j] )
{
if(' ' != ext_data[i] && ' ' != ext_data[i + 1])
{
ctx->out[10 + j].outPR[0] = ext_data[i];
ctx->out[10 + j].outPR[1] = ',';
ctx->out[10 + j].outPR[2] = ext_data[i + 1];
}
/*ctx->save.outPR[10+j][0] = ext_data[i];
ctx->save.outPR[10+j][1] = ',';
ctx->save.outPR[10+j][2] = ext_data[i+1];*/
if(0 != memcmp(ctx->out[10 + j].outPR, ctx->save.outPR[10 + j], 3))
{
//mod
printf("2+%d+%s\n", j + 1, ctx->save.outPR[10 + j]);
if('0' == ctx->save.outPR[10 + j][0] || '1' == ctx->save.outPR[10 + j][0])
{
memcpy(ctx->out[10 + j].outPR, ctx->save.outPR[10 + j], 1);
memset(sendbuf, 0, 128);
sprintf((char *)sendbuf, "SET-OUT-MOD:%c:%s::%c;",
j + EXT_2_OUT_LOWER,
"000000",
ctx->save.outPR[10 + j][0]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, j + EXT_2_OUT_LOWER, "000000");
}
}
}
}
}
else if((EXT_3_MGR_PRI == ext_sid) || (EXT_3_MGR_RSV == ext_sid))
{
for(i = 0, j = 0; i < 20 && j < 10; i += 2, j++)
{
if( EXT_OUT16 == ctx->extBid[2][j] ||
EXT_OUT32 == ctx->extBid[2][j] ||
EXT_OUT16S == ctx->extBid[2][j] ||
EXT_OUT32S == ctx->extBid[2][j] )
{
if(' ' != ext_data[i] && ' ' != ext_data[i + 1])
{
ctx->out[20 + j].outPR[0] = ext_data[i];
ctx->out[20 + j].outPR[1] = ',';
ctx->out[20 + j].outPR[2] = ext_data[i + 1];
}
/*ctx->save.outPR[20+j][0] = ext_data[i];
ctx->save.outPR[20+j][1] = ',';
ctx->save.outPR[20+j][2] = ext_data[i+1];*/
if(0 != memcmp(ctx->out[20 + j].outPR, ctx->save.outPR[20 + j], 3))
{
if((j + EXT_3_OUT_LOWER) >= ':')//except ':;'
{
slotid = j + EXT_3_OUT_LOWER + 2;
}
else
{
slotid = j + EXT_3_OUT_LOWER;
}
//mod
printf("3+%d+%s\n", j + 1, ctx->save.outPR[20 + j]);
if('0' == ctx->save.outPR[20 + j][0] || '1' == ctx->save.outPR[20 + j][0])
{
memcpy(ctx->out[20 + j].outPR, ctx->save.outPR[20 + j], 1);
memset(sendbuf, 0, 128);
sprintf((char *)sendbuf, "SET-OUT-MOD:%c:%s::%c;",
slotid,
"000000",
ctx->save.outPR[20 + j][0]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, slotid, "000000");
}
}
}
}
}
else
{
return 0;
}
return 1;
}
/*
1 成功
0 失败
*/
u8_t ext_out_bid_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx)
{
if((EXT_1_MGR_PRI == ext_sid) || (EXT_1_MGR_RSV == ext_sid))
{
memcpy(ctx->extBid[0], ext_data, 14);
}
else if((EXT_2_MGR_PRI == ext_sid) || (EXT_2_MGR_RSV == ext_sid))
{
memcpy(ctx->extBid[1], ext_data, 14);
}
else if((EXT_3_MGR_PRI == ext_sid) || (EXT_3_MGR_RSV == ext_sid))
{
//printf("<ext_out_bid_trpt>:%s\n",ext_data);
memcpy(ctx->extBid[2], ext_data, 14);
}
else
{
return 0;
}
return 1;
}
/*
1 成功
0 失败
*/
u8_t ext_mgr_pr_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx)
{
if((EXT_1_MGR_PRI == ext_sid) || (EXT_1_MGR_RSV == ext_sid))
{
ctx->mgr[0].mgrPR = ext_data[0] - '0';
ctx->mgr[1].mgrPR = ext_data[1] - '0';
}
else if((EXT_2_MGR_PRI == ext_sid) || (EXT_2_MGR_RSV == ext_sid))
{
ctx->mgr[2].mgrPR = ext_data[0] - '0';
ctx->mgr[3].mgrPR = ext_data[1] - '0';
}
else if((EXT_3_MGR_PRI == ext_sid) || (EXT_3_MGR_RSV == ext_sid))
{
ctx->mgr[4].mgrPR = ext_data[0] - '0';
ctx->mgr[5].mgrPR = ext_data[1] - '0';
}
else
{
return 0;
}
return 1;
}
/*
1 成功
0 失败
*/
u8_t ext_out_alm_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx)
{
int i, j;
u8_t tmp[7];
if((EXT_1_MGR_PRI == ext_sid) || (EXT_1_MGR_RSV == ext_sid))
{
for(i = 0, j = 0; i < 30 && j < 10; i += 4, j++)
{
memset(tmp, 0, 7);
tmp[0] = 0x40 | (ext_data[i + 0] & 0x0F);
tmp[1] = 0x40 | ((ext_data[i + 1] & 0x03) << 2) | ((ext_data[i + 0] >> 4) & 0x03);
tmp[2] = 0x40 | ((ext_data[i + 1] >> 2) & 0x0F);
tmp[3] = 0x40 | (ext_data[i + 2] & 0x0F);
tmp[4] = 0x40 | ((ext_data[i + 3] & 0x03) << 2) | ((ext_data[i + 2] >> 4) & 0x03);
tmp[5] = 0x40 |((ext_data[i + 3] >> 2) & 0x0F);
memcpy(ctx->out[j].outAlm, tmp, 6);
}
}
else if((EXT_2_MGR_PRI == ext_sid) || (EXT_2_MGR_RSV == ext_sid))
{
for(i = 0, j = 0; i < 30 && j < 10; i += 4, j++)
{
memset(tmp, 0, 7);
tmp[0] = 0x40 | (ext_data[i + 0] & 0x0F);
tmp[1] = 0x40 | ((ext_data[i + 1] & 0x03) << 2) | ((ext_data[i + 0] >> 4) & 0x03);
tmp[2] = 0x40 | ((ext_data[i + 1] >> 2) & 0x0F);
tmp[3] = 0x40 | (ext_data[i + 2] & 0x0F);
tmp[4] = 0x40 | ((ext_data[i + 3] & 0x03) << 2) | ((ext_data[i + 2] >> 4) & 0x03);
tmp[5] = 0x40 |((ext_data[i + 3] >> 2) & 0x0F);
memcpy(ctx->out[10 + j].outAlm, tmp, 6);
}
}
else if((EXT_3_MGR_PRI == ext_sid) || (EXT_3_MGR_RSV == ext_sid))
{
for(i = 0, j = 0; i < 30 && j < 10; i += 4, j++)
{
memset(tmp, 0, 7);
tmp[0] = 0x40 | (ext_data[i + 0] & 0x0F);
tmp[1] = 0x40 | ((ext_data[i + 1] & 0x03) << 2) | ((ext_data[i + 0] >> 4) & 0x03);
tmp[2] = 0x40 | ((ext_data[i + 1] >> 2) & 0x0F);
tmp[3] = 0x40 | (ext_data[i + 2] & 0x0F);
tmp[4] = 0x40 | ((ext_data[i + 3] & 0x03) << 2) | ((ext_data[i + 2] >> 4) & 0x03);
tmp[5] = 0x40 |((ext_data[i + 3] >> 2) & 0x0F);
memcpy(ctx->out[20 + j].outAlm, tmp, 6);
}
}
else
{
return 0;
}
return 1;
}
/*
1 成功
0 失败
*/
u8_t ext_out_ver_trpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx)
{
//drv
if('m' == ext_sid)
{
memcpy(ctx->drv[0].drvVer, ext_data, 22);
printf("%s\n", ctx->drv[0].drvVer);
}
else if('n' == ext_sid)
{
memcpy(ctx->drv[1].drvVer, ext_data, 22);
}
//mgr
else if(EXT_1_MGR_PRI == ext_sid)
{
memcpy(ctx->mgr[0].mgrVer, ext_data, 22);
}
else if(EXT_1_MGR_RSV == ext_sid)
{
memcpy(ctx->mgr[1].mgrVer, ext_data, 22);
}
else if(EXT_2_MGR_PRI == ext_sid)
{
memcpy(ctx->mgr[2].mgrVer, ext_data, 22);
}
else if(EXT_2_MGR_RSV == ext_sid)
{
memcpy(ctx->mgr[3].mgrVer, ext_data, 22);
}
else if(EXT_3_MGR_PRI == ext_sid)
{
memcpy(ctx->mgr[4].mgrVer, ext_data, 22);
}
else if(EXT_3_MGR_RSV == ext_sid)
{
memcpy(ctx->mgr[5].mgrVer, ext_data, 22);
}
//out
else if((ext_sid >= EXT_1_OUT_LOWER) && (ext_sid <= EXT_1_OUT_UPPER))
{
memcpy(ctx->out[ext_sid - EXT_1_OUT_OFFSET].outVer, ext_data, 22);
}
else if((ext_sid >= EXT_2_OUT_LOWER) && (ext_sid <= EXT_2_OUT_UPPER))
{
if(',' == ext_sid)
{
memcpy(ctx->out[ext_sid - EXT_2_OUT_OFFSET].outVer, ext_data + 1, 22);
}
else
{
memcpy(ctx->out[ext_sid - EXT_2_OUT_OFFSET].outVer, ext_data, 22);
}
}
else if((ext_sid >= EXT_3_OUT_LOWER) && (ext_sid <= EXT_3_OUT_UPPER))
{
if(ext_sid < 58)//except ':;'
{
memcpy(ctx->out[ext_sid - EXT_3_OUT_OFFSET].outVer, ext_data, 22);
}
else
{
memcpy(ctx->out[ext_sid - EXT_3_OUT_OFFSET - 2].outVer, ext_data, 22);
}
}
else
{
return 0;
}
return 1;
}
<file_sep>/MCPU/mcp_save.c
#include <sys/ipc.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/msg.h>
#include <signal.h>
#include <sys/ioctl.h>
#include <arpa/inet.h>
#include <net/if.h>
#include <net/if_arp.h>
#include <net/route.h>
#include <ctype.h>
#include "ext_global.h"
#include "ext_crpt.h"
#include "ext_trpt.h"
#include "mcp_main.h"
#include "mcp_process.h"
#include "mcp_def.h"
#include "mcp_save_struct.h"
#include "mcp_set_struct.h"
//#include "memwatch.h"
//#define ErrorOK 0
/*
*********************************************************************************************************
* Function: int save_gb_rcv_mode(char slot, unsigned char *parg)
* Descript: gbtpv2 alarm infomation.
* Parameters: - slot
* - parg :the point of input data.
* Return: - 0 : ok
* - 1 : error
*
*********************************************************************************************************
*/
int save_gb_rcv_mode(char slot, unsigned char *parg)
{
/*<acmode>*/
//int len;
if(slot == GBTP1_SLOT)
{
memset(rpt_content.slot_q.gb_acmode, 0, 4);
memcpy(rpt_content.slot_q.gb_acmode, parg, strlen(parg));
}
else if(slot == GBTP2_SLOT)
{
memset(rpt_content.slot_r.gb_acmode, 0, 4);
memcpy(rpt_content.slot_r.gb_acmode, parg, strlen(parg));
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_gb_work_mode(char slot, unsigned char *parg)
{
/*<pos_mode>*/
//int len;
if(slot == GBTP1_SLOT)
{
memcpy(rpt_content.slot_q.gb_pos_mode, parg, 4);
}
else if(slot == GBTP2_SLOT)
{
memcpy(rpt_content.slot_r.gb_pos_mode, parg, 4);
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
/*
*********************************************************************************************************
* Function: int save_gb_mask(char slot, unsigned char *parg)
* Descript: gbtpv2 enble setting event.
* Parameters: - slot
* - parg :the point of input data.
* Return: - 0 : ok
* - 1 : error
*
*********************************************************************************************************
*/
int save_gb_mask(char slot, unsigned char *parg)
{
int num;//, i, len
num = (int)(slot - 'a');
if((parg[0]!='0') && (parg[0]!='1'))
return 1;
if(slot == GBTP1_SLOT)
{
if(slot_type[num] != 'v')
{
memcpy(rpt_content.slot_q.gb_mask, parg, 2);
rpt_content.slot_q.gb_mask[2] = '\0';
}
else
{
rpt_content.slot_q.staen = parg[0];
//rpt_content.slot_q.gb_mask[0] = parg[0];
//rpt_content.slot_q.gb_mask[1] = '\0';
}
}
else if(slot == GBTP2_SLOT)
{
if(slot_type[num] != 'v')
{
memcpy(rpt_content.slot_r.gb_mask, parg, 2);
rpt_content.slot_r.gb_mask[2] = '\0';
}
else
{
rpt_content.slot_r.staen = parg[0];
//rpt_content.slot_r.gb_mask[0] = parg[0];
//rpt_content.slot_r.gb_mask[1] = '\0';
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_gb_bdzb(char slot, unsigned char *parg)
{
int len = 0;
if((strncmp1(parg, "BJ54", 4) != 0) && (strncmp1(parg, "CGS2000", 7) != 0))
{
return 1;
}
len = strlen(parg);
if(slot == GBTP1_SLOT)
{
memcpy(rpt_content.slot_q.bdzb, parg, len );
}
else if(slot == GBTP2_SLOT)
{
memcpy(rpt_content.slot_r.bdzb, parg, len);
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_rb_msmode(char slot, unsigned char *parg)
{
unsigned char buf[4];
/*<msmode>::=MAIN|STAN*/
if(slot == RB1_SLOT)
{
memcpy(rpt_content.slot_o.rb_msmode, parg, 4);
get_out_lev(0, 0);
memcpy(buf, rpt_content.slot_o.tod_buf, 3);
//printf("<save_rb_msmode>:%s\n",buf);
send_tod(buf, 'o');
}
else if(slot == RB2_SLOT)
{
memcpy(rpt_content.slot_p.rb_msmode, parg, 4);
get_out_lev(0, 0);
memcpy(buf, rpt_content.slot_p.tod_buf, 3);
//printf("<save_rb_msmode>:%s\n",buf);
send_tod(buf, 'p');
}
#if DEBUG_NET_INFO
if(slot == RB1_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_o.rb_msmode);
}
}
else if(slot == RB2_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_p.rb_msmode);
}
}
#endif
return 0;
}
int save_rb_src(char slot, unsigned char *parg)
{
/*<ref>::= y */
//int flag_lev=0;
//int i;
if(slot == RB1_SLOT)
{
rpt_content.slot_o.rb_ref = parg[0];
get_out_lev(0, 0);
}
else if(slot == RB2_SLOT)
{
rpt_content.slot_p.rb_ref = parg[0];
get_out_lev(0, 0);
}
/*#if DEBUG_NET_INFO
if(slot==RB1_SLOT)
{
printf("%s:<%c>%s#\n",NET_SAVE,slot,rpt_content.slot_o.rb_ref);
}
else if(slot==RB2_SLOT)
{
printf("%s:<%c>%s#\n",NET_SAVE,slot,rpt_content.slot_p.rb_ref);
}
#endif*/
return 0;
}
int save_rb_prio(char slot, unsigned char *parg)
{
/*<priority>::=xxxxxxxx*/
if(slot == RB1_SLOT)
{
memcpy(rpt_content.slot_o.rb_prio, parg, 8);
}
else if(slot == RB2_SLOT)
{
memcpy(rpt_content.slot_p.rb_prio, parg, 8);
}
#if DEBUG_NET_INFO
if(slot == RB1_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_o.rb_prio);
}
}
else if(slot == RB2_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_p.rb_prio);
}
}
#endif
return 0;
}
int save_rb_mask(char slot, unsigned char *parg)
{
/*<mask>::=xxxxxxxx*/
if(slot == RB1_SLOT)
{
memcpy(rpt_content.slot_o.rb_mask, parg, 8);
}
else if(slot == RB2_SLOT)
{
memcpy(rpt_content.slot_p.rb_mask, parg, 8);
}
#if DEBUG_NET_INFO
if(slot == RB1_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_o.rb_mask);
}
}
else if(slot == RB2_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_p.rb_mask);
}
}
#endif
return 0;
}
int save_rf_tzo(char slot, unsigned char *parg)
{
/*<we>,<out>*/
if(slot == RB1_SLOT)
{
memcpy(rpt_content.slot_o.rf_tzo, parg, 3);
}
else if(slot == RB2_SLOT)
{
memcpy(rpt_content.slot_p.rf_tzo, parg, 3);
}
#if DEBUG_NET_INFO
if(slot == RB1_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_o.rf_tzo);
}
}
else if(slot == RB2_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_p.rf_tzo);
}
}
#endif
return 0;
}
int save_rb_dey_chg(char slot, unsigned char *parg)
{
/**/
int num;
num = (int)(parg[0] - 0x31);
if(slot == RB1_SLOT)
{
memset(rpt_content.slot_o.rf_dey[num], 0, 9);
memcpy(rpt_content.slot_o.rf_dey[num], &parg[2], strlen(&parg[2]));
}
else if(slot == RB2_SLOT)
{
memset(rpt_content.slot_p.rf_dey[num], 0, 9);
memcpy(rpt_content.slot_p.rf_dey[num], &parg[2], strlen(&parg[2]));
}
#if DEBUG_NET_INFO
if(slot == RB1_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_o.rf_dey[num]);
}
}
else if(slot == RB2_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_p.rf_dey[num]);
}
}
#endif
return 0;
}
int save_rb_sta_chg(char slot, unsigned char *parg)
{
/*<trmode>::=TRCK|HOLD|FATR|TEST|FREE*/
if(slot == RB1_SLOT)
{
memcpy(rpt_content.slot_o.rb_trmode, parg, 4);
get_out_lev(0, 0);
}
else if(slot == RB2_SLOT)
{
memcpy(rpt_content.slot_p.rb_trmode, parg, 4);
get_out_lev(0, 0);
}
#if DEBUG_NET_INFO
if(slot == RB1_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_o.rb_trmode);
}
}
else if(slot == RB2_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_p.rb_trmode);
}
}
#endif
return 0;
}
int save_rb_leap_chg(char slot, unsigned char *parg)
{
/*<leap>::=xx*/
if(slot == RB1_SLOT)
{
memcpy(rpt_content.slot_o.rb_leap, parg, 2);
}
else if(slot == RB2_SLOT)
{
memcpy(rpt_content.slot_p.rb_leap, parg, 2);
}
#if DEBUG_NET_INFO
if(slot == RB1_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_o.rb_leap);
}
}
else if(slot == RB2_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_o.rb_leap);
}
}
#endif
return 0;
}
int save_rb_leap_flg(char slot, unsigned char *parg)
{
/*<leapmask>::=xxxxx*/
if(slot == RB1_SLOT)
{
memcpy(rpt_content.slot_o.rb_leapmask, parg, 5);
}
else if(slot == RB2_SLOT)
{
memcpy(rpt_content.slot_p.rb_leapmask, parg, 5);
}
#if DEBUG_NET_INFO
if(slot == RB1_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_o.rb_leapmask);
}
}
else if(slot == RB2_SLOT)
{
if(print_switch == 0)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, rpt_content.slot_p.rb_leapmask);
}
}
#endif
return 0;
}
int save_tod_sta(char slot, unsigned char *parg)
{
int num;
// unsigned char tmp[17];
// memset(tmp,'\0',sizeof(unsigned char )*17);
num = (int)(slot - 'a');
if(slot_type[num] != 'I')
{
//
send_online_framing(num, 'I');
slot_type[num] = 'I';
}
memcpy(rpt_content.slot[num].tp16_tod, parg, 3);
memcpy(rpt_content.slot[num].tp16_en, &parg[4], 16);
//rpt_content.slot[num].tp16_en[16]='\0';
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
//printf("<save_tod_sta>:%s\n",parg);
return 0;
}
int save_out_lev(char slot, unsigned char *parg)
{
int num, len;
if(slot >= 'a' && slot <= 'n')
{
num = (int)(slot - 'a');
len = strlen(parg);
if(2 == len)
{
memcpy(rpt_content.slot[num].out_lev, parg, 2);
}
else
{
return(1);
}
}
else
{
if(2 == strlen(parg))
{
if(0 == ext_out_ssm_crpt(0, slot, parg, &gExtCtx))
{
return 1;
}
}
else
{
if(0 == ext_out_ssm_trpt(slot, parg, &gExtCtx))
{
return 1;
}
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
//
//
//
int save_out_typ(char slot, unsigned char *parg)
{
int num, len;
if(slot >= 'a' && slot <= 'n')
{
num = (int)(slot - 'a');
len = strlen(parg);
if(16 == len)
{
memcpy(rpt_content.slot[num].out_typ, parg, 16);
}
else
{
return(1);
}
}
else
{
if(16 == strlen(parg))
{
if(0 == ext_out_signal_crpt(0, slot, parg, &gExtCtx))
{
return 1;
}
}
else
{
if(0 == ext_out_signal_trpt(slot, parg, &gExtCtx))
{
return 1;
}
}
}
//
//!OUT32S ADD.
//
//printf("out32s type change:%d,%s#\n", num,parg);
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_ptp_net(char slot, unsigned char *parg)
{
int num, port;
int i, j, i1, i2, i3, i4, i5, i6, len;
j = i1 = i2 = i3 = i4 = i5 = i6 = 0;
num = (int)(slot - 'a');
port = (int)(parg[0] - 0x31);
len = strlen(parg);
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
if(j == 5)
{
i5 = i + 1;
}
if(j == 6)
{
i6 = i + 1;
}
}
}
if(i6 == 0)
{
return (1);
}
//memcpy(rpt_content.slot[num].ptp_net[port],parg,99);
// flag=i2-i1-1;
//if(flag!=0)
if(parg[i1] != ',')
{
memcpy(rpt_content.slot[num].ptp_ip[port], &parg[i1], i2 - i1 - 1);
}
if(parg[i2] != ',')
{
memcpy(rpt_content.slot[num].ptp_mac[port], &parg[i2], i3 - i2 - 1);
}
if(parg[i3] != ',')
{
memcpy(rpt_content.slot[num].ptp_gate[port], &parg[i3], i4 - i3 - 1);
}
if(parg[i4] != ',')
{
memcpy(rpt_content.slot[num].ptp_mask[port], &parg[i4], i5 - i4 - 1);
}
if(parg[i5] != ',')
{
memcpy(rpt_content.slot[num].ptp_dns1[port], &parg[i5], i6 - i5 - 1);
}
if(parg[i6] != '\0')
{
memcpy(rpt_content.slot[num].ptp_dns2[port], &parg[i6], len - i6);
}
//printf("\n\n\nLUOHAO------22,Get: rpt_content.slot[%d].ptp_ip[%d]=%s\n\n\n",num,port,rpt_content.slot[num].ptp_ip[port]);
#if DEBUG_NET_INFO
if(print_switch == 0)
printf("%s:%s,%s,%s,%s,%s,%s#\n", NET_SAVE, rpt_content.slot[num].ptp_ip[port],
rpt_content.slot[num].ptp_mac[port], rpt_content.slot[num].ptp_gate[port],
rpt_content.slot[num].ptp_mask[port], rpt_content.slot[num].ptp_dns1[port],
rpt_content.slot[num].ptp_dns2[port]);
#endif
return 0;
}
int save_ptp_mod(char slot, unsigned char *parg)
{
int i, j, i1, i2, i3, i4, i5, i6, i7, i8, i9, i10, i11, len, i12;
int num, port;
j = i1 = i2 = i3 = i4 = i5 = i6 = i7 = i8 = i9 = i10 = i11 = i12 = 0;
num = (int)(slot - 'a');
port = (int)(parg[0] - 0x31);
len = strlen(parg);
//parg[len] = '\0';
//printf("ptp recv %s\n",parg);
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
if(j == 5)
{
i5 = i + 1;
}
if(j == 6)
{
i6 = i + 1;
}
if(j == 7)
{
i7 = i + 1;
}
if(j == 8)
{
i8 = i + 1;
}
if(j == 9)
{
i9 = i + 1;
}
if(j == 10)
{
i10 = i + 1;
}
if(j == 11)
{
i11 = i + 1;
}
if(j == 12)
{
i12 = i + 1;
}
}
}
if(i11 == 0)
{
return (1);
}
if((parg[i1] == '1') || (parg[i1] == '0') )
{
rpt_content.slot[num].ptp_en[port] = parg[i1];
}
if((parg[i2] == '1') || (parg[i2] == '0'))
{
rpt_content.slot[num].ptp_delaytype[port] = parg[i2];
}
if((parg[i3] == '1') || (parg[i3] == '0'))
{
rpt_content.slot[num].ptp_multicast[port] = parg[i3];
}
if((parg[i4] == '1') || (parg[i4] == '0'))
{
rpt_content.slot[num].ptp_enp[port] = parg[i4];
}
if((parg[i5] == '1') || (parg[i5] == '0'))
{
rpt_content.slot[num].ptp_step[port] = parg[i5];
}
if(parg[i6] != ',')
{
memcpy(rpt_content.slot[num].ptp_sync[port], &parg[i6], i7 - i6 - 1);
}
if(parg[i7] != ',')
{
memcpy(rpt_content.slot[num].ptp_announce[port], &parg[i7], i8 - i7 - 1);
}
if(parg[i8] != ',')
{
memcpy(rpt_content.slot[num].ptp_delayreq[port], &parg[i8], i9 - i8 - 1);
}
if(parg[i9] != ',')
{
memcpy(rpt_content.slot[num].ptp_pdelayreq[port], &parg[i9], i10 - i9 - 1);
}
if(parg[i10] != ',')
{
memset(rpt_content.slot[num].ptp_delaycom[port],0,9);
memcpy(rpt_content.slot[num].ptp_delaycom[port], &parg[i10], i11 - i10 - 1);
}
if((parg[i11] == '1') || (parg[i11] == '0'))
{
rpt_content.slot[num].ptp_linkmode[port] = parg[i11];
}
if((parg[i12] == '1') || (parg[i12] == '0'))
{
rpt_content.slot[num].ptp_out[port] = parg[i12];
}
//printf("\n\n\nLUOHAO------33,Get: rpt_content.slot[%d].ptp_multicast[%d]=%d\n\n\n",num,port,rpt_content.slot[num].ptp_multicast[port]);
/*rpt_content.slot[num].ptp_out[port]='1';*/
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s,<%c>#\n", NET_SAVE, parg, rpt_content.slot[num].ptp_linkmode[port]);
}
#endif
return 0;
}
int save_ptp_mtc(char slot, unsigned char *parg)
{
int i, j, i_ip, i_prio1, i_prio2;
int len, num, port;
i = j = i_ip = i_prio1 = i_prio2 = 0;
num = (int)(slot - 'a');
port = (int)(parg[0] - 0x31);
len = strlen(parg);
//
//!
//
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i_ip = i + 1;
}
if(j == 2)
{
i_prio1 = i + 1;
}
if(j == 3)
{
i_prio2 = i + 1;
}
}
}
if(i_ip == 0)
{
return (1);
}
if(parg[i_ip] != ',')
{
memset(&rpt_ptp_mtc.ptp_mtc_ip[num][port][0],'\0',9);
memcpy(&rpt_ptp_mtc.ptp_mtc_ip[num][port][0], &parg[i_ip], 8);
}
if(parg[i_prio1] != ',')
{
memset(&rpt_ptp_mtc.ptp_prio1[num][port][0], 0, 5);
for (i = i_prio1; parg[i] != ','; i++)
{
memcpy(&rpt_ptp_mtc.ptp_prio1[num][port][i - i_prio1], &parg[i], 1);
}
}
if(parg[i_prio2] != ',')
{
memset(&rpt_ptp_mtc.ptp_prio2[num][port][0], 0, 5);
for (i = i_prio2; parg[i] != '\0'; i++) //
{
memcpy(&rpt_ptp_mtc.ptp_prio2[num][port][i - i_prio2], &parg[i], 1);
}
}
return 0;
}
int save_ptp_mtc16(char slot, unsigned char *parg)
{
int i, j, i_ip, i_inx;
int len, num, port,str_len;
i = j = i_inx = i_ip = 0;
num = (int)(slot - 'a');
port = (int)(parg[0] - 0x31);
len = strlen(parg);
str_len = 0;
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i_inx = i + 1;
}
if(j == 2)
{
i_ip = i + 1;
}
}
}
if(i_inx == 0)
return 1;
if(port < 0 || port > 3)
return 1;
if(parg[i_inx] == '1')
{
//一帧上报8个IP地址,每个IP 8个字节,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX
if((len - 4) < 8 || (len - 4) > 71)
return 1;
memset(&rpt_ptp_mtc.ptp_mtc_ip[num][port][0],'\0',sizeof(rpt_ptp_mtc.ptp_mtc_ip[num][port]));
memcpy(&rpt_ptp_mtc.ptp_mtc_ip[num][port][0], &parg[i_ip], len - 4);
}
else if(parg[i_inx] == '2')
{
//一帧上报8个IP地址,每个IP 8个字节,,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX
if((len - 4) < 8 || (len - 4) > 72)
return 1;
str_len = strlen(rpt_ptp_mtc.ptp_mtc_ip[num][port]);
//一帧上报8个IP地址,每个IP 8个字节,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX,XXXXXXXX
if(str_len > 71)
return 1;
memcpy(&rpt_ptp_mtc.ptp_mtc_ip[num][port][0] + str_len , &parg[i_ip], len - 4);
}
else
{
return 1;
}
return 0;
}
//
//! 2017/6/16 添加PTP闰秒事件上报CC7
//
int save_ptp_sta(char slot, unsigned char *parg)
{
int num, i;
int len;
num = (int)(slot - 'a');
len = strlen(parg);
if((0 == len)||(2 < len))
{
return 1;
}
i = atoi(parg);
if((i < 0)||(i > 99))
return 1;
if(slot_type[num] == 'v' || slot_type[num] == 'x' )
{
if(slot == GBTP1_SLOT)
{
memset(rpt_content.slot_q.leapnum,0,sizeof(rpt_content.slot_q.leapnum));
memcpy(rpt_content.slot_q.leapnum, &parg[0], len);
}
else
{
memset(rpt_content.slot_r.leapnum,0,sizeof(rpt_content.slot_r.leapnum));
memcpy(rpt_content.slot_r.leapnum, &parg[0], len);
}
}
else
{
memset(rpt_content.slot[num].ntp_leap,0,sizeof(rpt_content.slot[num].ntp_leap));
memcpy(rpt_content.slot[num].ntp_leap, &parg[0], len);
}
//printf("ptp leap %d,%s\n",num,parg);
return 0;
}
int save_Leap_sta(char slot, unsigned char *parg)
{
int num, i;
int len;
num = (int)(slot - 'a');
len = strlen(parg);
if((0 == len)||(2 < len))
{
return 1;
}
i = atoi(parg);
if((i < 0)||(i > 99))
return 1;
if(slot_type[num] == 'v' || slot_type[num] == 'x')
{
if(slot == GBTP1_SLOT)
{
memset(rpt_content.slot_q.leapnum,0,sizeof(rpt_content.slot_q.leapnum));
memcpy(rpt_content.slot_q.leapnum, &parg[0], len);
}
else
{
memset(rpt_content.slot_r.leapnum,0,sizeof(rpt_content.slot_r.leapnum));
memcpy(rpt_content.slot_r.leapnum, &parg[0], len);
}
}
//printf("ptp leap %d,%s\n",num,parg);
return 0;
}
int save_ptp_dom(char slot, unsigned char *parg)
{
int num,port ;
int len,i_dom,i,j ;
num = port = 0;
i = j = len = i_dom = 0;
num = (int)(slot - 'a');
port = (int)(parg[0] - 0x31);
len =strlen(parg);
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i_dom = i+1;
}
}
}
if(i_dom != 2)
return (1);
if((port < 0)||(port > 4))
return 1;
if((len == 0)||(len > 5))
return 1;
if(parg[i_dom] != ',')
{
memset(&rpt_ptp_mtc.ptp_dom[num][port][0],0,4);
memcpy(&rpt_ptp_mtc.ptp_dom[num][port][0], &parg[i_dom],len - i_dom );
}
return 0;
}
/**********************/
int save_gb_sta(char slot, unsigned char *parg)
{
int i, j, i1, i2, i3, i4, len, i5, i6;
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
i5 = 0;
i6 = 0;
len = strlen(parg);
/*<pos_mode>,<delay>,<elevation>,<format>,<acmode>
POST,20,8888,IRIGA,GPS*/
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
if(j == 5)
{
i5 = i + 1;
}
if(j == 6)
{
i6 = i + 1;
}
}
}
//if(i6==0)/***************/
// return (1);
if(slot == GBTP1_SLOT)
{
if(parg[0] != ',')
{
memcpy(rpt_content.slot_q.gb_pos_mode, parg, 4);
}
if(parg[i2] != ',')
{
memcpy(rpt_content.slot_q.gb_elevation, &parg[i2], i3 - i2 - 1);
}
if(parg[i3] != ',')
{
memcpy(rpt_content.slot_q.gb_format, &parg[i3], i4 - i3 - 1);
}
if(parg[i4] != ',')
{
memset(rpt_content.slot_q.gb_acmode, 0, 4);
memcpy(rpt_content.slot_q.gb_acmode, &parg[i4], i5 - i4 - 1);
}
if(parg[i5] == '0' || parg[i5] == '1' || parg[i5] == '2')
{
rpt_content.slot_q.gb_sta = parg[i5];
}
if(parg[i6] == '0')
{
memcpy(rpt_content.slot_q.bdzb, "BJ54", 4);
}
else if(parg[i6] == '1')
{
memcpy(rpt_content.slot_q.bdzb, "CGS2000", 7);
}
}
else if(slot == GBTP2_SLOT)
{
if(parg[0] != ',')
{
memcpy(rpt_content.slot_r.gb_pos_mode, parg, 4);
}
if(parg[i2] != ',')
{
memcpy(rpt_content.slot_r.gb_elevation, &parg[i2], i3 - i2 - 1);
}
if(parg[i3] != ',')
{
memcpy(rpt_content.slot_r.gb_format, &parg[i3], i4 - i3 - 1);
}
if(parg[i4] != ',')
{
memset(rpt_content.slot_r.gb_acmode, 0, 4);
memcpy(rpt_content.slot_r.gb_acmode, &parg[i4], i5 - i4 - 1);
}
if(parg[i5] == '0' || parg[i5] == '1' || parg[i5] == '2')
{
rpt_content.slot_r.gb_sta = parg[i5];
}
if(parg[i6] == '0')
{
memcpy(rpt_content.slot_r.bdzb, "BJ54", 4);
}
else if(parg[i6] == '1')
{
memcpy(rpt_content.slot_r.bdzb, "CGS2000", 7);
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
/*
*********************************************************************************************************
* Function: int save_gbtp_sta(char slot, unsigned char *parg)
* Descript: gbtpv2 status infomation.
* Parameters: - slot
* - parg :the point of input data.
* Return: - 0 : ok
* - 1 : error
*
*********************************************************************************************************
*/
int save_gbtp_sta(char slot, unsigned char *parg)
{
int i, j, i1, i2, i3, i4, len, i5;//, i6
int usError;
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
i5 = 0;
usError = 0;
//i6 = 0;
len = strlen(parg);
/*<saten>,<acmode>,<linkstatus>,<pos_mode>,<rec_type>,<rec_ver>
POST,20,8888,IRIGA,GPS*/
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
if(j == 5)
{
i5 = i + 1;
}
}
}
if(i5 == 0)
return 1;
if(slot == GBTP1_SLOT)
{
if((parg[0] == '0')||(parg[0] == '1'))
{
rpt_content.slot_q.staen = parg[0];
}
if(parg[i1] != ',')
{
//judge the len lt 4.
usError = ((i2 - i1 - 1) < sizeof(rpt_content.slot_q.gb_acmode)) ? 0:1;
if(usError)
return 1;
//excete memset and memcpy,save data.
memset(rpt_content.slot_q.gb_acmode, 0, 4);
memcpy(rpt_content.slot_q.gb_acmode, &parg[i1], i2 - i1 - 1);
}
if(parg[i2] == '0' || parg[i2] == '1' || parg[i2] == '2')
{
rpt_content.slot_q.gb_sta = parg[i2];
}
if(parg[i3] != ',')
{
//judge the len et 4.
usError = ((i4 - i3 - 1) < sizeof(rpt_content.slot_q.gb_pos_mode)) ? 0:1;
if(usError)
return 1;
//excete memset and memcpy,save data.
memcpy(rpt_content.slot_q.gb_pos_mode,&parg[i3], i4 - i3 - 1);
rpt_content.slot_q.gb_pos_mode[i4 - i3 - 1] = '\0';
}
if(parg[i4] != ',')
{
//judge the len et 11.
usError = ((i5 - i4 - 1) < sizeof(rpt_content.slot_q.rec_type)) ? 0:1;
if(usError)
return 1;
//excete memset and memcpy,save data.
memcpy(rpt_content.slot_q.rec_type, &parg[i4], i5 - i4 - 1);
rpt_content.slot_q.rec_type[i5 - i4 - 1] = '\0';
}
if(parg[i5] != '\0')
{
//judge the len et 9.
usError = ((len - i5) < sizeof(rpt_content.slot_q.rec_ver)) ? 0:1;
if(usError)
return 1;
//excete memset and memcpy,save data.
memcpy(rpt_content.slot_q.rec_ver, &parg[i5], len -i5);
rpt_content.slot_q.rec_ver[len - i5] = '\0';
}
}
else if(slot == GBTP2_SLOT)
{
if((parg[0] == '0')||(parg[0] == '1'))
{
rpt_content.slot_r.staen = parg[0];
}
if(parg[i1] != ',')
{
//judge the len lt 4.
usError = ((i2 - i1 - 1) < sizeof(rpt_content.slot_r.gb_acmode)) ? 0:1;
if(usError)
return 1;
//excete memset and memcpy,save data.
memset(rpt_content.slot_r.gb_acmode, 0, 4);
memcpy(rpt_content.slot_r.gb_acmode, &parg[i1], i2 - i1 - 1);
}
if(parg[i2] == '0' || parg[i2] == '1' || parg[i2] == '2')
{
rpt_content.slot_r.gb_sta = parg[i2];
}
if(parg[i3] != ',')
{
//judge the len et 4.
usError = ((i4 - i3 - 1) < sizeof(rpt_content.slot_r.gb_pos_mode)) ? 0:1;
if(usError)
return 1;
//excete memset and memcpy,save data.
memcpy(rpt_content.slot_r.gb_pos_mode,&parg[i3], i4 - i3 - 1);
rpt_content.slot_r.gb_pos_mode[i4 - i3 - 1] = '\0';
}
if(parg[i4] != ',')
{
//judge the len et 10.
usError = ((i5 - i4 - 1) < sizeof(rpt_content.slot_r.rec_type)) ? 0:1;
if(usError)
return 1;
//excete memset and memcpy,save data.
memcpy(rpt_content.slot_r.rec_type, &parg[i4], i5 - i4 - 1);
rpt_content.slot_r.rec_type[i5 - i4 - 1] = '\0';
}
if(parg[i5] != '\0')
{
//judge the len et 9.
usError = ((len - i5) < sizeof(rpt_content.slot_r.rec_ver)) ? 0:1;
if(usError)
return 1;
//excete memset and memcpy,save data.
memcpy(rpt_content.slot_r.rec_ver, &parg[i5], len -i5);
rpt_content.slot_r.rec_ver[len - i5] = '\0';
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
/*
*********************************************************************************************************
* Function: int save_gbtp_delay(char slot, unsigned char *parg)
* Descript: gbtpIII delay.
* Parameters: - slot
* - parg :the point of input data.
* Return: - 0 : ok
* - 1 : error
*
*********************************************************************************************************
*/
int is_digit(unsigned char *parg,unsigned char len)
{
int i;
int usError;
usError = 0;
for(i = 0; i < len; i++)
{
if(i == 0)
{
if(parg[0] != '-')
{
if(parg[0] < 0x30 || parg[0] > 0x39)
{
usError = 1;
break;
}
}
}
else if(parg[i] < 0x30 || parg[i] > 0x39)
{
usError = 1;
break;
}
}
return usError;
}
int save_gbtp_delay(char slot, unsigned char *parg)
{
int len;
int usError;
usError = 0;
len = strlen(parg);
/*<delay>; -32768 ~ 32767 */
if(len <= 0 || len > 6)
return 1;
/*judge if the parg is or digit*/
if(is_digit(parg, len))
return 1;
if(slot == GBTP1_SLOT)
{
//memset(rpt_content.slot_q.delay, '\0', sizeof(rpt_content.slot_q.delay));
memcpy(rpt_content.slot_q.delay, &parg[0], len);
rpt_content.slot_q.delay[len] = '\0';
}
else if(slot == GBTP2_SLOT)
{
//memset(rpt_content.slot_r.delay, '\0', sizeof(rpt_content.slot_r.delay));
memcpy(rpt_content.slot_r.delay, &parg[0], len);
rpt_content.slot_r.delay[len] = '\0';
}
printf("%s:gn_dly,%s#\n", NET_SAVE, parg);
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
/*GBTPIII 1PPS out delay,range of -400000 ~ 400000,unit 2.5ns*/
int save_pps_delay(char slot, unsigned char *parg)
{
int len;
int usError;
usError = 0;
len = strlen(parg);
/*<delay>; -400000 ~ 400000 */
if(len <= 0 || len > 10)
return 1;
/*judge if the parg is or digit*/
if(is_digit(parg, len))
return 1;
if(slot == GBTP1_SLOT)
{
//memset(rpt_content.slot_q.delay, '\0', sizeof(rpt_content.slot_q.delay));
memcpy(rpt_content.slot_q.pps_out_delay, &parg[0], len);
rpt_content.slot_q.pps_out_delay[len] = '\0';
}
else if(slot == GBTP2_SLOT)
{
//memset(rpt_content.slot_r.delay, '\0', sizeof(rpt_content.slot_r.delay));
memcpy(rpt_content.slot_r.pps_out_delay, &parg[0], len);
rpt_content.slot_r.pps_out_delay[len] = '\0';
}
printf("%s:pps_dly,%s#\n", NET_SAVE, parg);
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_gb_ori(char slot, unsigned char *parg)
{
if(slot == GBTP1_SLOT)
{
memcpy(rpt_content.slot_q.gb_ori, parg, strlen(parg));
}
else if(slot == GBTP2_SLOT)
{
memcpy(rpt_content.slot_r.gb_ori, parg, strlen(parg));
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
/*********************************************************************************************************
* Function: int save_gbtp_pst(char slot, unsigned char *parg)
* Descript: gbtpv2 post time infomation.
* Parameters: - slot
* - parg :the point of input data.
* Return: - 0 : ok
* - 1 : error
*
*********************************************************************************************************/
int save_gbtp_pst(char slot, unsigned char *parg)
{
int sLen = 0;
//string length .
sLen = ((0 == strlen(parg))||(strlen(parg) > 70))? 0:strlen(parg);
if(!sLen)
return 1;
//copy the data.
if(slot == GBTP1_SLOT)
{
memcpy(rpt_content.slot_q.gb_ori, parg, sLen);
rpt_content.slot_q.gb_ori[sLen] = '\0';
}
else if(slot == GBTP2_SLOT)
{
memcpy(rpt_content.slot_r.gb_ori, parg, sLen);
rpt_content.slot_r.gb_ori[sLen] = '\0';
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_gb_rev(char slot, unsigned char *parg)
{
if(slot == GBTP1_SLOT)
{
if(parg[0] == 'G')
{
memset(rpt_content.slot_q.gb_rev_g, '\0', 256);
memcpy(rpt_content.slot_q.gb_rev_g, parg, strlen(parg));
}
else if(parg[0] == 'B')
{
memset(rpt_content.slot_q.gb_rev_b, '\0', 256);
memcpy(rpt_content.slot_q.gb_rev_b, parg, strlen(parg));
}
}
else if(slot == GBTP2_SLOT)
{
if(parg[0] == 'G')
{
memset(rpt_content.slot_r.gb_rev_g, '\0', 256);
memcpy(rpt_content.slot_r.gb_rev_g, parg, strlen(parg));
}
else if(parg[0] == 'B')
{
memset(rpt_content.slot_r.gb_rev_b, '\0', 256);
memcpy(rpt_content.slot_r.gb_rev_b, parg, strlen(parg));
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
/*
*********************************************************************************************************
* Function: int save_gbtp_info(char slot, unsigned char *parg)
* Descript: gbtpv2 status infomation.
* Parameters: - slot
* - parg :the point of input data.
* Return: - 0 : ok
* - 1 : error
*
*********************************************************************************************************
*/
int save_gbtp_info(char slot, unsigned char *parg)
{
if(slot == GBTP1_SLOT)
{
if(parg[0] == 'G' && parg[1] == 'P' && parg[2] == 'S')
{
memset(rpt_content.slot_q.gb_rev_g, '\0', 256);
memcpy(rpt_content.slot_q.gb_rev_g, parg, strlen(parg));
}
else if(parg[0] == 'B' && parg[1] == 'D')
{
memset(rpt_content.slot_q.gb_rev_b, '\0', 256);
memcpy(rpt_content.slot_q.gb_rev_b, parg, strlen(parg));
}
else if(parg[0] == 'G' && parg[1] == 'L'&& parg[2] == 'O')
{
memset(rpt_content.slot_q.gb_rev_glo, '\0', 256);
memcpy(rpt_content.slot_q.gb_rev_glo, parg, strlen(parg));
}
else if(parg[0] == 'G' && parg[1] == 'A'&& parg[2] == 'L')
{
memset(rpt_content.slot_q.gb_rev_gal, '\0', 256);
memcpy(rpt_content.slot_q.gb_rev_gal, parg, strlen(parg));
}
}
else if(slot == GBTP2_SLOT)
{
if(parg[0] == 'G' && parg[1] == 'P' && parg[2] == 'S')
{
memset(rpt_content.slot_r.gb_rev_g, '\0', 256);
memcpy(rpt_content.slot_r.gb_rev_g, parg, strlen(parg));
}
else if(parg[0] == 'B' && parg[1] == 'D')
{
memset(rpt_content.slot_r.gb_rev_b, '\0', 256);
memcpy(rpt_content.slot_r.gb_rev_b, parg, strlen(parg));
}
else if(parg[0] == 'G' && parg[1] == 'L'&& parg[2] == 'O')
{
memset(rpt_content.slot_r.gb_rev_glo, '\0', 256);
memcpy(rpt_content.slot_r.gb_rev_glo, parg, strlen(parg));
}
else if(parg[0] == 'G' && parg[1] == 'A'&& parg[2] == 'L')
{
memset(rpt_content.slot_r.gb_rev_gal, '\0', 256);
memcpy(rpt_content.slot_r.gb_rev_gal, parg, strlen(parg));
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_gb_tod(char slot, unsigned char *parg)
{
unsigned char sendbuf[SENDBUFSIZE];
unsigned char ctag[6] = "000000";
memset(sendbuf, '\0', SENDBUFSIZE);
if(slot == GBTP1_SLOT)
{
memcpy(rpt_content.slot_q.gb_tod, parg, 3);
}
else if(slot == GBTP2_SLOT)
{
memcpy(rpt_content.slot_r.gb_tod, parg, 3);
}
/** SET-TOD-STA:<ctag>::<ms>,<ppssta>,<sourcetype>; ***/
sprintf((char *)sendbuf, "SET-TOD-STA:%s::%d,%s;", ctag, (slot == GBTP1_SLOT) ? 0 : 1, parg);
//printf("<save_gb_tod>:%s\n",sendbuf);
if(slot_type[15] == 'K' || slot_type[15] == 'R')
{
sendtodown(sendbuf, (unsigned char)RB2_SLOT);
}
if(slot_type[14] == 'K' || slot_type[14] == 'R')
{
sendtodown(sendbuf, (unsigned char)RB1_SLOT);
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_gb_alm(char slot, unsigned char *parg)
{
int num;
unsigned char tmp[12];
memset(tmp, '\0', 12);
num = (int)(slot - 'a');
rpt_alm_to_client(slot, parg);
sprintf((char *)tmp, "\"%c,%c,%s\"", slot, slot_type[num], parg);
if(slot == GBTP1_SLOT)
{
memset(alm_sta[16],'\0',14);
memcpy(alm_sta[16], tmp, strlen(tmp));
}
else if(slot == GBTP2_SLOT)
{
memset(alm_sta[17],'\0',14);
memcpy(alm_sta[17], tmp, strlen(tmp));
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
/*
*********************************************************************************************************
* Function: int save_gbtp_alm(char slot, unsigned char *parg)
* Descript: gbtpv2 alarm infomation.
* Parameters: - slot
* - parg :the point of input data.
* Return: - 0 : ok
* - 1 : error
*
*********************************************************************************************************
*/
int save_gbtp_alm(char slot, unsigned char *parg)
{
int num;
unsigned char tmp[12];
memset(tmp, '\0', 12);
num = (int)(slot - 'a');
rpt_alm_to_client(slot, parg);
sprintf((char *)tmp, "\"%c,%c,%s\"", slot, slot_type[num], parg);
if(slot == GBTP1_SLOT)
{
memset(alm_sta[16],'\0',14);
memcpy(alm_sta[16], tmp, strlen(tmp));
}
else if(slot == GBTP2_SLOT)
{
memset(alm_sta[17],'\0',14);
memcpy(alm_sta[17], tmp, strlen(tmp));
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_sy_ver(char slot, unsigned char *parg)
{
int num, len;
int i, j, i1, i2, i3,i4,i5;
unsigned char mcu_ver[7];
unsigned char fpga_ver[7];
unsigned char pcb_ver[7];
unsigned char temp[40];
if( (('m' == slot) && (EXT_DRV == slot_type[12]) ) ||
(('n' == slot) && (EXT_DRV == slot_type[13])) ||
(EXT_1_MGR_PRI == slot) || (EXT_1_MGR_RSV == slot) ||
(EXT_2_MGR_PRI == slot) || (EXT_2_MGR_RSV == slot) ||
(EXT_3_MGR_PRI == slot) || (EXT_3_MGR_RSV == slot) ||
(slot >= EXT_1_OUT_LOWER && slot <= EXT_1_OUT_UPPER) ||
(slot >= EXT_2_OUT_LOWER && slot <= EXT_2_OUT_UPPER) ||
(slot >= EXT_3_OUT_LOWER && slot <= EXT_3_OUT_UPPER) )
{
if(0 == ext_out_ver_trpt(slot, parg, &gExtCtx))
{
return 1;
}
}
else
{
//printf("main-----ver :%s slot :%d\n",parg,slot);
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 =0;
i5 = 0;
len = strlen(parg);
if(strncmp1(parg, "MCU", 3) != 0) //
{
if(slot == RB1_SLOT)
{
memcpy(rpt_content.slot_o.sy_ver, parg, len);
}
else if(slot == RB2_SLOT)
{
memcpy(rpt_content.slot_p.sy_ver, parg, len);
}
else if(slot == GBTP1_SLOT)
{
memset(rpt_content.slot_q.sy_ver, '\0', sizeof(rpt_content.slot_q.sy_ver));
memcpy(rpt_content.slot_q.sy_ver, parg, len);
/*MCU,V01.00,FPGA,V01.00,CPLD,V01.00,PCB,V01.00,*/
/*V01.00,V01.00,V01.00,<sta>*/
if(rpt_content.slot_q.gb_sta == '0' || rpt_content.slot_q.gb_sta == '1' || rpt_content.slot_q.gb_sta == '2')
{
rpt_content.slot_q.sy_ver[21] = rpt_content.slot_q.gb_sta;
rpt_content.slot_q.sy_ver[22] = '\0';
}
else
{
rpt_content.slot_q.sy_ver[21] = '\0';
}
}
else if(slot == GBTP2_SLOT)
{
memset(rpt_content.slot_r.sy_ver, '\0', sizeof(rpt_content.slot_r.sy_ver));
memcpy(rpt_content.slot_r.sy_ver, parg, len);
if(rpt_content.slot_r.gb_sta == '0' || rpt_content.slot_r.gb_sta == '1' || rpt_content.slot_r.gb_sta == '2')
{
rpt_content.slot_r.sy_ver[21] = rpt_content.slot_r.gb_sta;
rpt_content.slot_r.sy_ver[22] = '\0';
}
else
{
rpt_content.slot_r.sy_ver[21] = '\0';
}
}
else
{
num = (int)(slot - 'a');
if((num >= 0) && (num < 14))
{
memcpy(rpt_content.slot[num].sy_ver, parg, len);
//printf("--%s--\n", rpt_content.slot[num].sy_ver);
}
}
}
else
{
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
i4 = i+1;
if(j == 5)
i5 = i+1;
}
}
if(i3 == 0)
{
return 1;
}
/*MCU,V01.00,FPGA,V01.00,PCB,V01.00*/
memset(pcb_ver,0,7);
memset(mcu_ver, 0, 7);
memcpy(mcu_ver, &parg[i1], i2 - i1 - 1);
memset(fpga_ver, 0, 7);
if(i4 == 0)
{
memcpy(fpga_ver, &parg[i3], len - i3);
sprintf((char *)temp, "%s,%s,,", mcu_ver, fpga_ver);
}
else
{
memcpy(fpga_ver, &parg[i3], i4 - i3-1);
memcpy(pcb_ver, &parg[i5], len - i5);
sprintf((char *)temp, "%s,%s,,%s", mcu_ver, fpga_ver,pcb_ver);
}
len = strlen(temp);
if(slot == RB1_SLOT)
{
memcpy(rpt_content.slot_o.sy_ver, temp, len);
}
else if(slot == RB2_SLOT)
{
memcpy(rpt_content.slot_p.sy_ver, temp, len);
}
else if(slot == GBTP1_SLOT)
{
memset(rpt_content.slot_q.sy_ver, '\0', sizeof(rpt_content.slot_q.sy_ver));
memcpy(rpt_content.slot_q.sy_ver, temp, len);
}
else if(slot == GBTP2_SLOT)
{
memset(rpt_content.slot_r.sy_ver, '\0', sizeof(rpt_content.slot_r.sy_ver));
memcpy(rpt_content.slot_r.sy_ver, temp, len);
}
else
{
num = (int)(slot - 'a');
if((num >= 0) && (num < 14))
{
memset(rpt_content.slot[num].sy_ver, '\0', sizeof(rpt_content.slot[num].sy_ver));
memcpy(rpt_content.slot[num].sy_ver, temp, len);
}
}
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_ch1andch3dph(char slot, unsigned char *parg)
{
int i, j, i1, i2, i3, i4, i5, i6, i7,i8, len;
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
i5 = 0;
i6 = 0;
i7 = 0;
i8 = 0;
len = strlen(parg);
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
if(j == 5)
{
i5 = i + 1;
}
if(j == 6)
{
i6 = i + 1;
}
if(j == 7)
{
i7 = i + 1;
}
if(j == 8)
{
i8 = i + 1;
}
}
}
if(i8 == 0)
{
return 1;
}
if(slot == RB1_SLOT)
{
if(parg[i1] != ',')
{
memset(rpt_content.slot_o.rb_phase[0], 0, 9);
memcpy(rpt_content.slot_o.rb_phase[0], &parg[i1], i2 - i1 - 1);
}
else
{
memset(rpt_content.slot_o.rb_phase[0], 0, 9);
}
if(parg[i3] != ',')
{
memset(rpt_content.slot_o.rb_phase[1], 0, 9);
memcpy(rpt_content.slot_o.rb_phase[1], &parg[i3], i4 - i3 - 1);
}
else
{
memset(rpt_content.slot_o.rb_phase[1], 0, 9);
}
}
else if(slot == RB2_SLOT)
{
if(parg[i1] != ',')
{
memset(rpt_content.slot_p.rb_phase[0], 0, 9);
memcpy(rpt_content.slot_p.rb_phase[0], &parg[i1], i2 - i1 - 1);
}
else
{
memset(rpt_content.slot_p.rb_phase[0], 0, 9);
}
if(parg[i3] != ',')
{
memset(rpt_content.slot_p.rb_phase[1], 0, 9);
memcpy(rpt_content.slot_p.rb_phase[1], &parg[i3], i4 - i3 - 1);
}
else
{
memset(rpt_content.slot_p.rb_phase[1], 0, 9);
}
}
return 0;
}
int save_rx_dph(char slot, unsigned char *parg)
{
//
//!new defined.
int i;
int j,i1,i2,i3,i4,i5,i6,i7,i8,len;
unsigned char tmp[SENDBUFSIZE];
memset(tmp, '\0', SENDBUFSIZE);
//
//!new initial.
len=i= 0;
j=i1=i2=i3=i4=i5=i6=i7=i8=0;
len = strlen(parg);
for(i =0;i<len;i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1) i1 = i+1;
if(j == 2) i2 = i+1;
if(j == 3) i3 = i+1;
if(j == 4) i4 = i+1;
if(j == 5) i5 = i+1;
if(j == 6) i6 = i+1;
if(j == 7) i7 = i+1;
if(j == 8) i8 = i+1;
}
}
if(i8 == 0)
return 1;
get_time_fromnet();
if(slot == RB1_SLOT)
{
sprintf((char *)tmp, "\"%c,%s,%s,%s\"%c%c%c%c%c", slot, parg, mcp_date, mcp_time, CR, LF, SP, SP, SP);
memcpy(rpt_content.slot_o.rb_inp[flag_i_o], tmp, strlen(tmp));
flag_i_o++;
if(flag_i_o == 10)
{
flag_i_o = 0;
}
//
//!new record #17 #18 #1 time 2# time 2# fre 1#
// #17 #18 2mhz 2mb
if(parg[0] != ',')
strncpynew(rpt_content.slot_o.rb_ph[0],&parg[0],i1-1);
else
memset(rpt_content.slot_o.rb_ph[0],'\0',10);
if(parg[i2] != ',')
strncpynew(rpt_content.slot_o.rb_ph[1],&parg[i2],i3-i2-1);
else
memset(rpt_content.slot_o.rb_ph[1],'\0',10);
if(parg[i1] != ',')
strncpynew(rpt_content.slot_o.rb_ph[2],&parg[i1],i2-i1-1);
else
memset(rpt_content.slot_o.rb_ph[2],'\0',10);
if(parg[i3] != ',')
strncpynew(rpt_content.slot_o.rb_ph[3],&parg[i3],i4-i3-1);
else
memset(rpt_content.slot_o.rb_ph[3],'\0',10);
if(parg[i5] != ',')
strncpynew(rpt_content.slot_o.rb_ph[4],&parg[i5],i6-i5-1);
else
memset(rpt_content.slot_o.rb_ph[4],'\0',10);
if(parg[i7] != ',')
strncpynew(rpt_content.slot_o.rb_ph[5],&parg[i7],i8-i7-1);
else
memset(rpt_content.slot_o.rb_ph[5],'\0',10);
if(parg[i8] != '\0')
strncpynew(rpt_content.slot_o.rb_ph[6],&parg[i8],len-i8);
}
if(slot == RB2_SLOT)
{
sprintf((char *)tmp, "\"%c,%s,%s,%s\"%c%c%c%c%c", slot, parg, mcp_date, mcp_time, CR, LF, SP, SP, SP);
memcpy(rpt_content.slot_p.rb_inp[flag_i_p], tmp, strlen(tmp));
flag_i_p++;
if(flag_i_p == 10)
{
flag_i_p = 0;
}
//
//!new record #17 #18 #1 time 2# time 2# fre 1#
// #17 #18 2mhz 2mb
if(parg[0] != ',')
strncpynew(rpt_content.slot_p.rb_ph[0],&parg[0],i1-1);
else
memset(rpt_content.slot_p.rb_ph[0],'\0',10);
if(parg[i2] != ',')
strncpynew(rpt_content.slot_p.rb_ph[1],&parg[i2],i3-i2-1);
else
memset(rpt_content.slot_p.rb_ph[1],'\0',10);
if(parg[i1] != ',')
strncpynew(rpt_content.slot_p.rb_ph[2],&parg[i1],i2-i1-1);
else
memset(rpt_content.slot_p.rb_ph[2],'\0',10);
if(parg[i3] != ',')
strncpynew(rpt_content.slot_p.rb_ph[3],&parg[i3],i4-i3-1);
else
memset(rpt_content.slot_p.rb_ph[3],'\0',10);
if(parg[i4] != ',')
strncpynew(rpt_content.slot_p.rb_ph[4],&parg[i4],i5-i4-1);
else
memset(rpt_content.slot_p.rb_ph[4],'\0',10);
if(parg[i6] != ',')
strncpynew(rpt_content.slot_p.rb_ph[5],&parg[i6],i7-i6-1);
else
memset(rpt_content.slot_p.rb_ph[5],'\0',10);
if(parg[i8] != '\0')
strncpynew(rpt_content.slot_p.rb_ph[6],&parg[i8],len-i8);
}
save_ch1andch3dph(slot,parg);
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_rf_src(char slot, unsigned char *parg)
{
int i, j, i1, i2, i3, i4, i5, len;
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
i5 = 0;
len = strlen(parg);
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
if(j == 5)
{
i5 = i + 1;
}
}
}
if(i5 == 0)
{
return 1;
}
if(slot == RB1_SLOT)
{
if(parg[0] != ',')
{
rpt_content.slot_o.rb_ref = parg[0];
get_out_lev(0, 0);
}
if(parg[i1] != ',')
{
memcpy(rpt_content.slot_o.rb_prio, &parg[i1], i2 - i1 - 1);
}
if(parg[i2] != ',')
{
memcpy(rpt_content.slot_o.rb_mask, &parg[i2], i3 - i2 - 1);
}
if(parg[i3] != ',')
{
memcpy(rpt_content.slot_o.rb_leapmask, &parg[i3], i4 - i3 - 1);
}
if(parg[i4] != ',')
{
memcpy(rpt_content.slot_o.rb_leap, &parg[i4], i5 - i4 - 1);
}
if(parg[i5] != '\0')
{
rpt_content.slot_o.rb_leaptag = parg[i5];
}
}
else if(slot == RB2_SLOT)
{
if(parg[0] != ',')
{
rpt_content.slot_p.rb_ref = parg[0];
get_out_lev(0, 0);
}
if(parg[i1] != ',')
{
memcpy(rpt_content.slot_p.rb_prio, &parg[i1], i2 - i1 - 1);
}
if(parg[i2] != ',')
{
memcpy(rpt_content.slot_p.rb_mask, &parg[i2], i3 - i2 - 1);
}
if(parg[i3] != ',')
{
memcpy(rpt_content.slot_p.rb_leapmask, &parg[i3], i4 - i3 - 1);
}
if(parg[i4] != ',')
{
memcpy(rpt_content.slot_p.rb_leap, &parg[i4], i5 - i4 - 1);
}
if(parg[i5] != '\0')
{
rpt_content.slot_p.rb_leaptag = parg[i5];
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
if(slot == RB1_SLOT)
printf("%s:<%c>%s,%s,%s,%s#\n", NET_SAVE, slot, rpt_content.slot_o.rb_prio,
rpt_content.slot_o.rb_mask, rpt_content.slot_o.rb_leapmask,
rpt_content.slot_o.rb_leap);
else if(slot == RB2_SLOT)
printf("%s:<%c>%s,%s,%s,%s#\n", NET_SAVE, slot, rpt_content.slot_p.rb_prio,
rpt_content.slot_p.rb_mask, rpt_content.slot_p.rb_leapmask,
rpt_content.slot_p.rb_leap);
}
#endif
return 0;
}
int save_rb_tod(char slot, unsigned char *parg)
{
/** SET-TOD-STA:<ctag>::<ms>,<ppssta>,<sourcetype>; **/
unsigned char sendbuf[SENDBUFSIZE];
//unsigned char *ctag="000000";
//char send_slot;
//int i;
int value = 0;
char tmp_buf[64];
//printf("<save_rb_tod>:%s\n",parg);
memset(sendbuf, '\0', SENDBUFSIZE);
if(slot == RB1_SLOT)
{
if(strncmp1(rpt_content.slot_o.rb_msmode, "MAIN", 4) == 0)
{
value = 0;
}
//else if(strncmp1(rpt_content.slot_o.rb_msmode,"STBY",4)==0)
// value=1;
else
{
return 0;
}
}
else if(slot == RB2_SLOT)
{
if(strncmp1(rpt_content.slot_p.rb_msmode, "MAIN", 4) == 0)
{
value = 0;
}
//else if(strncmp1(rpt_content.slot_p.rb_msmode,"STBY",4)==0)
// value=1;
else
{
return 0;
}
}
else
{
return 0;
}
//modified by zhanghui 2013-9-10 15:50:12
memset(tmp_buf, 0, 64);
memcpy(tmp_buf, parg, strlen(parg));
//printf("<save_rb_tod>:%s\n",parg);
send_tod(tmp_buf, slot);
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_rf_dey(char slot, unsigned char *parg)
{
int i, j, i1, i2, i3, i4, i5, i6, i7, len;
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
i5 = 0;
i6 = 0;
i7 = 0;
len = strlen(parg);
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
if(j == 5)
{
i5 = i + 1;
}
if(j == 6)
{
i6 = i + 1;
}
if(j == 7)
{
i7 = i + 1;
}
}
}
if(i7 == 0)
{
return 1;
}
if(slot == RB1_SLOT)
{
if(parg[0] != ',')
{
memset(rpt_content.slot_o.rf_dey[0], 0, 9);
memcpy(rpt_content.slot_o.rf_dey[0], &parg[0], i1 - 1);
}
if(parg[i1] != ',')
{
memset(rpt_content.slot_o.rf_dey[1], 0, 9);
memcpy(rpt_content.slot_o.rf_dey[1], &parg[i1], i2 - i1 - 1);
}
if(parg[i2] != ',')
{
memset(rpt_content.slot_o.rf_dey[2], 0, 9);
memcpy(rpt_content.slot_o.rf_dey[2], &parg[i2], i3 - i2 - 1);
}
if(parg[i3] != ',')
{
memset(rpt_content.slot_o.rf_dey[3], 0, 9);
memcpy(rpt_content.slot_o.rf_dey[3], &parg[i3], i4 - i3 - 1);
}
if(parg[i4] != ',')
{
memset(rpt_content.slot_o.rf_dey[4], 0, 9);
memcpy(rpt_content.slot_o.rf_dey[4], &parg[i4], i5 - i4 - 1);
}
if(parg[i5] != ',')
{
memset(rpt_content.slot_o.rf_dey[5], 0, 9);
memcpy(rpt_content.slot_o.rf_dey[5], &parg[i5], i6 - i5 - 1);
}
if(parg[i6] != ',')
{
memset(rpt_content.slot_o.rf_dey[6], 0, 9);
memcpy(rpt_content.slot_o.rf_dey[6], &parg[i6], i7 - i6 - 1);
}
if(parg[i7] != '\0')
{
memset(rpt_content.slot_o.rf_dey[7], 0, 9);
memcpy(rpt_content.slot_o.rf_dey[7], &parg[i7], len - i7);
}
}
else if(slot == RB2_SLOT)
{
if(parg[0] != ',')
{
memset(rpt_content.slot_p.rf_dey[0], 0, 9);
memcpy(rpt_content.slot_p.rf_dey[0], &parg[0], i1 - 1);
}
if(parg[i1] != ',')
{
memset(rpt_content.slot_p.rf_dey[1], 0, 9);
memcpy(rpt_content.slot_p.rf_dey[1], &parg[i1], i2 - i1 - 1);
}
if(parg[i2] != ',')
{
memset(rpt_content.slot_p.rf_dey[2], 0, 9);
memcpy(rpt_content.slot_p.rf_dey[2], &parg[i2], i3 - i2 - 1);
}
if(parg[i3] != ',')
{
memset(rpt_content.slot_p.rf_dey[3], 0, 9);
memcpy(rpt_content.slot_p.rf_dey[3], &parg[i3], i4 - i3 - 1);
}
if(parg[i4] != ',')
{
memset(rpt_content.slot_p.rf_dey[4], 0, 9);
memcpy(rpt_content.slot_p.rf_dey[4], &parg[i4], i5 - i4 - 1);
}
if(parg[i5] != ',')
{
memset(rpt_content.slot_p.rf_dey[5], 0, 9);
memcpy(rpt_content.slot_p.rf_dey[5], &parg[i5], i6 - i5 - 1);
}
if(parg[i6] != ',')
{
memset(rpt_content.slot_p.rf_dey[6], 0, 9);
memcpy(rpt_content.slot_p.rf_dey[6], &parg[i6], i7 - i6 - 1);
}
if(parg[i7] != '\0')
{
memset(rpt_content.slot_p.rf_dey[7], 0, 9);
memcpy(rpt_content.slot_p.rf_dey[7], &parg[i7], len - i7);
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_rx_sta(char slot, unsigned char *parg)
{
int i, j, i1, i2, len, i3, i4, i5;
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', sizeof(unsigned char) * SENDBUFSIZE);
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
i5 = 0;
len = strlen(parg);
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
if(j == 5)
{
i5 = i + 1;
}
}
}
if(i5 == 0)
{
return 1;
}
if(slot == RB1_SLOT)
{
if(parg[0] != ',')
{
memcpy(rpt_content.slot_o.rb_typework, &parg[0], i1 - 1);
}
if(parg[i1] != ',')
{
memcpy(rpt_content.slot_o.rb_msmode, &parg[i1], i2 - i1 - 1);
get_out_lev(0, 0);
}
if(parg[i2] != ',')
{
memcpy(rpt_content.slot_o.rb_trmode, &parg[i2], i3 - i2 - 1);
get_out_lev(0, 0);
}
if(parg[i3] != ',')
{
if(parg[i3] != conf_content.slot_u.fb)
{
sprintf((char *)sendbuf, "SET-RB-FB:000000::%c;", conf_content.slot_u.fb);
sendtodown(sendbuf, RB1_SLOT);
}
}
if(parg[i4] != ',')
{
if((parg[i4] < '@') || (parg[i4] > 'E'))
{}
else
{
rpt_content.slot_o.rb_sa = parg[i4];
}
}
if(parg[i5] != '\0')
{
if((parg[i5] < 'a') || (parg[i5] > 'g'))
{}
else
{
rpt_content.slot_o.rb_tl = parg[i5];
}
}
}
else if(slot == RB2_SLOT)
{
if(parg[0] != ',')
{
memcpy(rpt_content.slot_p.rb_typework, &parg[0], i1 - 1);
}
if(parg[i1] != ',')
{
memcpy(rpt_content.slot_p.rb_msmode, &parg[i1], i2 - i1 - 1);
get_out_lev(0, 0);
}
if(parg[i2] != ',')
{
memcpy(rpt_content.slot_p.rb_trmode, &parg[i2], i3 - i2 - 1);
get_out_lev(0, 0);
}
if(parg[i3] != ',')
{
if(parg[i3] != conf_content.slot_u.fb)
{
sprintf((char *)sendbuf, "SET-RB-FB:000000::%c;", conf_content.slot_u.fb);
sendtodown(sendbuf, RB2_SLOT);
}
}
if(parg[i4] != ',')
{
if((parg[i4] < '@') || (parg[i4] > 'E'))
{
}
else
{
rpt_content.slot_p.rb_sa = parg[i4];
}
}
if(parg[i5] != '\0')
{
if((parg[i5] < 'a') || (parg[i5] > 'g'))
{
}
else
{
rpt_content.slot_p.rb_tl = parg[i5];
}
}
}
get_out_lev(0, 0);
#if DEBUG_NET_INFO
if(print_switch == 0)
{
if(slot == RB1_SLOT)
printf("%s:<%c>%s,%s,%s#\n", NET_SAVE, slot, rpt_content.slot_o.rb_typework,
rpt_content.slot_o.rb_msmode, rpt_content.slot_o.rb_trmode);
else if(slot == RB2_SLOT)
printf("%s:<%c>%s,%s,%s#\n", NET_SAVE, slot, rpt_content.slot_p.rb_typework,
rpt_content.slot_p.rb_msmode, rpt_content.slot_p.rb_trmode);
}
#endif
return 0;
}
int save_rx_alm(char slot, unsigned char *parg)
{
unsigned char tmp[14];
int num;
unsigned char buf[4];
memset(tmp, '\0', 14);
num = (int)(slot - 'a');
rpt_alm_to_client(slot, parg);
get_out_lev(0, 0);
sprintf((char *)tmp, "\"%c,%c,%s\"", slot, slot_type[num], parg);
if(slot == RB1_SLOT)
{
memcpy(alm_sta[14], tmp, strlen(tmp));
strncpynew(buf, rpt_content.slot_o.tod_buf, 3);
//printf("<save_rx_alm>:%s\n",buf);
send_tod(buf, slot);
}
else if(slot == RB2_SLOT)
{
memcpy(alm_sta[15], tmp, strlen(tmp));
strncpynew(buf, rpt_content.slot_p.tod_buf, 3);
//printf("<save_rx_alm>:%s\n",buf);
send_tod(buf, slot);
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
if(slot == RB1_SLOT)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, alm_sta[14]);
}
else if(slot == RB2_SLOT)
{
printf("%s:<%c>%s#\n", NET_SAVE, slot, alm_sta[15]);
}
}
#endif
return 0;
}
int save_tp_alm(char slot, unsigned char *parg)
{
int num;
unsigned char tmp[14];
memset(tmp, '\0', 14);
rpt_alm_to_client(slot, parg);
sprintf((char *)tmp, "\"%c,I,%s\"", slot, parg);
num = (int)(slot - 'a');
memcpy(alm_sta[num], tmp, strlen(tmp));
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_out_alm(char slot, unsigned char *parg)
{
int num;
unsigned char tmp[14];
if (strlen(parg) > 14)
{
printf("\n\n\n! ! ! para overlength : %s\n\n\n", __func__);
return -1;
}
if(slot >= 'a' && slot <= 'w')
{
memset(tmp, '\0', 14);
num = (int)(slot - 'a');
rpt_alm_to_client(slot, parg);
sprintf((char *)tmp, "\"%c,%c,%s\"", slot, slot_type[num], parg);
memcpy(alm_sta[num], tmp, strlen(tmp));
}
else
{
if(0 == ext_out_alm_trpt(slot, parg, &gExtCtx))
{
return 1;
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
int save_ptp_alm(char slot, unsigned char *parg)
{
int num;
unsigned char tmp[14];
memset(tmp, '\0', 14);
rpt_alm_to_client(slot, parg);
num = (int)(slot - 'a');
sprintf((char *)tmp, "\"%c,%c,%s\"", slot, slot_type[num], parg);
memcpy(alm_sta[num], tmp, strlen(tmp));
//printf("PTP ALM %s \n",alm_sta[num]);
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
void JudgeAllowExtOnLine(const char slot, unsigned char *parg)
{
u8_t online;
u8_t flag;
online = gExtCtx.save.onlineSta;
if( (EXT_1_MGR_PRI == slot || EXT_1_MGR_RSV == slot)
&& ( (online & 0x01) == 0x00 ) )
{
printf("---1\n");
flag = 0;
}
else if( (EXT_2_MGR_PRI == slot || EXT_2_MGR_RSV == slot)
&& ( (online & 0x02) == 0x00 ) )
{
printf("---2\n");
flag = 0;
}
else if( (EXT_3_MGR_PRI == slot || EXT_3_MGR_RSV == slot)
&& ( (online & 0x04) == 0x00 ) )
{
printf("---3\n");
flag = 0;
}
else
{
flag = 1;
}
if(0 == flag)
{
memset(parg, 0, 14);
memset(parg, 'O', 14);
}
else
{
}
}
int save_ext_bid(char slot, unsigned char *parg)
{
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
JudgeAllowExtOnLine(slot, parg);
if(0 == ext_bpp_cfg(slot, parg, &gExtCtx))
{
return 1;
}
if(0 == ext_bid_cmp(slot, parg, &gExtCtx))
{
return 1;
}
if(0 == ext_out_bid_trpt(slot, parg, &gExtCtx))
{
return 1;
}
return 0;
}
int save_ext_mgr_pr(char slot, unsigned char *parg)
{
if(print_switch == 0)
{
printf("%s:%s#\n", NET_SAVE, parg);
}
if(0 == ext_mgr_pr_trpt(slot, parg, &gExtCtx))
{
return 1;
}
return 0;
}
int save_out_sta(char slot, unsigned char *parg)
{
int num;
if(slot >= 'a' && slot <= 'n')
{
num = (int)(slot - 'a');
if((parg[0] == '1') || (parg[0] == '0'))
{
rpt_content.slot[num].out_mode = parg[0];
}
else
{
return 1;
}
if((parg[2] == '1') || (parg[2] == '0'))
{
rpt_content.slot[num].out_sta = parg[2];
}
else
{
return 1;
}
//
//OUT32S ADD
//
//printf("out32s mod change:%d,%s\n",num,parg);
}
else
{
if(3 == strlen(parg))
{
if(0 == ext_out_mode_crpt(0, slot, parg, &gExtCtx))
{
return 1;
}
}
else
{
if(0 == ext_out_mode_trpt(slot, parg, &gExtCtx))
{
return 1;
}
}
}
return 0;
}
int save_out_prr(char slot, unsigned char *parg)
{
if(slot >= 'a' && slot <= 'n')
{
return 0;
}
else
{
if(0 == ext_out_prr_crpt(slot, parg, &gExtCtx))
{
return 1;
}
else
{
return 0;
}
}
}
int save_ref_prio(char slot, unsigned char *parg)
{
int num, i, len;
num = (int)(slot - 'a');
if(slot_type[num] != 'S')
{
//
send_online_framing(num, 'S');
slot_type[num] = 'S';
}
len = strlen(parg);
if(len == 9)
{
if(parg[0] == ',') //wu prio1
{
for(i = 0; i < 8; i++)
{
if((parg[i + 1] < 0x30) || (parg[i + 1] > 0x38))
{
return 1;
}
}
memcpy(rpt_content.slot[num].ref_prio2, &parg[1], 8);
}
else
{
for(i = 0; i < 8; i++)
{
if((parg[i] < 0x30) || (parg[i] > 0x38))
{
return 1;
}
}
memcpy(rpt_content.slot[num].ref_prio1, parg, 8);
}
}
else if(len == 17)
{
for(i = 0; i < 8; i++)
{
if((parg[i + 9] < 0x30) || (parg[i + 9] > 0x38))
{
return 1;
}
if((parg[i] < 0x30) || (parg[i] > 0x38))
{
return 1;
}
}
memcpy(rpt_content.slot[num].ref_prio2, &parg[9], 8);
memcpy(rpt_content.slot[num].ref_prio1, parg, 8);
}
else
{
return 1;
}
return 0;
}
int save_ref_mod(char slot, unsigned char *parg)
{
int num, len;
num = (int)(slot - 'a');
len = strlen(parg);
if(slot_type[num] != 'S')
{
//
send_online_framing(num, 'S');
slot_type[num] = 'S';
}
if(len == 5)
{
if(parg[0] == ',')
{
if(((parg[2] == '0') || (parg[2] == '1')) &&
((parg[4] > 0x2f) && (parg[4] < 0x39)))
{
memcpy(rpt_content.slot[num].ref_mod2, &parg[2], 3);
// send_ref_lev(num);
get_out_lev(0, 0);
}
else
{
return 1;
}
}
else
{
if(((parg[0] == '0') || (parg[0] == '1')) &&
((parg[2] > 0x2f) && (parg[2] < 0x39)))
{
memcpy(rpt_content.slot[num].ref_mod1, &parg[0], 3);
//send_ref_lev(num);
get_out_lev(0, 0);
}
else
{
return 1;
}
}
}
else if(len == 7)
{
if(((parg[0] == '0') || (parg[0] == '1')) &&
((parg[2] > 0x2f) && (parg[2] < 0x39)))
{}
else
{
return 1;
}
if(((parg[4] == '0') || (parg[4] == '1')) &&
((parg[6] > 0x2f) && (parg[6] < 0x39)))
{}
else
{
return 1;
}
memcpy(rpt_content.slot[num].ref_mod1, &parg[0], 3);
memcpy(rpt_content.slot[num].ref_mod2, &parg[4], 3);
//send_ref_lev(num);
get_out_lev(0, 0);
}
else
{
return 1;
}
return 0;
}
int save_ref_ph(char slot, unsigned char *parg)
{
int num, i, cnt, j1;
num = (int)(slot - 'a');
cnt = i = j1 = 0;
if(slot_type[num] != 'S')
{
//
send_online_framing(num, 'S');
slot_type[num] = 'S';
}
while(parg[i])
{
if(parg[i] == ',')
{
cnt++;
if(cnt == 1)
{
j1 = i + 1;
}
}
i++;
}
if(cnt != 8)
{
return 1;
}
memcpy(rpt_content.slot[num].ref_en, parg, 8);
memset(rpt_content.slot[num].ref_ph, 0, 128);
memcpy(rpt_content.slot[num].ref_ph, &parg[9], strlen(parg) - 9);
return 0;
}
int save_ref_sta(char slot, unsigned char *parg)
{
int num, i, len;
num = (int)(slot - 'a');
if(slot_type[num] != 'S')
{
//
send_online_framing(num, 'S');
slot_type[num] = 'S';
}
len = strlen(parg);
if(len != 17)
{
return 1;
}
for(i = 0; i < 8; i++)
{
if((parg[i] < '@') && (parg[i] > 'A'))
{
return 1;
}
}
for(i = 9; i < 8; i++)
{
if((parg[i] < 'a') && (parg[i] > 'g'))
{
return 1;
}
}
memcpy(rpt_content.slot[num].ref_sa, parg, 8);
memcpy(rpt_content.slot[num].ref_tl, &parg[9], 8);
//send_ref_lev(num);
get_out_lev(0, 0);
return 0;
}
int save_ref_tpy(char slot, unsigned char *parg)
{
int num, i;
num = (int)(slot - 'a');
if(slot_type[num] != 'S')
{
//
send_online_framing(num, 'S');
slot_type[num] = 'S';
}
for(i = 0; i < 8; i++)
{
if((parg[i] != '0') && (parg[i] != '1') && (parg[i] != '2'))
{
return 1;
}
}
for(i = 0; i < 8; i++)
{
if((parg[i + 9] != '0') && (parg[i + 9] != '1'))
{
return 1;
}
}
memcpy(rpt_content.slot[num].ref_typ1, parg, 8);
memcpy(rpt_content.slot[num].ref_typ2, &parg[9], 8);
return 0;
}
int save_ref_alm(char slot, unsigned char *parg)
{
int num;
unsigned char tmp[14];
memset(tmp, '\0', 14);
num = (int)(slot - 'a');
rpt_alm_to_client(slot, parg);
sprintf((char *)tmp, "\"%c,S,%s\"", slot, parg);
//printf("<save_ref_alm>:%s\n",tmp);
if((parg[0] >> 4) == 0x05)
{
if(num == 0) //ref1
{
memcpy(alm_sta[18], tmp, strlen(tmp));
}
else if(num == 1) //ref2
{
memcpy(alm_sta[19], tmp, strlen(tmp));
}
}
else
{
memcpy(alm_sta[num], tmp, strlen(tmp));
}
return 0;
}
int save_ref_en(char slot, unsigned char *parg)
{
int num, i;
num = (int)(slot - 'a');
if(slot_type[num] != 'S')
{
//
send_online_framing(num, 'S');
slot_type[num] = 'S';
}
for(i = 0; i < 8; i++)
{
if((parg[i] != '1') && (parg[i] != '0'))
{
return 1;
}
}
memcpy(rpt_content.slot[num].ref_en, parg, 8);
return 0;
}
int save_ref_ssm_en(char slot, unsigned char *parg)
{
int num, i;
num = (int)(slot - 'a');
if(slot_type[num] != 'S')
{
//
send_online_framing(num, 'S');
slot_type[num] = 'S';
}
for(i = 0; i < 8; i++)
{
if(parg[i] < '0' || parg[i] > '2')
{
return 1;
}
}
memcpy(rpt_content.slot[num].ref_ssm_en, parg, 8);
return 0;
}
int save_ref_sa(char slot, unsigned char *parg)
{
int num, i;
num = (int)(slot - 'a');
if(slot_type[num] != 'S')
{
//
send_online_framing(num, 'S');
slot_type[num] = 'S';
}
for(i = 0; i < 8; i++)
{
if((parg[i] < '@') && (parg[i] > 'E'))
{
return 1;
}
}
memcpy(rpt_content.slot[num].ref_sa, parg, 8);
return 0;
}
int save_ref_tl(char slot, unsigned char *parg)
{
int num, i;
num = (int)(slot - 'a');
if(slot_type[num] != 'S')
{
//
send_online_framing(num, 'S');
slot_type[num] = 'S';
}
for(i = 0; i < 8; i++)
{
if((parg[i] < 'a') && (parg[i] > 'g'))
{
return 1;
}
}
memcpy(rpt_content.slot[num].ref_tl, parg, 8);
//send_ref_lev(num);
get_out_lev(0, 0);
return 0;
}
int save_rs_en(char slot, unsigned char *parg)
{
int num, i;
num = (int)(slot - 'a');
/*if(slot_type[num]!='T')
{
//
send_online_framing(num,'T');
slot_type[num]='T';
}*/
for(i = 0; i < 4; i++)
{
if((parg[i] != '1') && (parg[i] != '0'))
{
return 1;
}
}
memcpy(rpt_content.slot[num].rs_en, parg, 4);
return 0;
}
int save_rs_tzo(char slot, unsigned char *parg)
{
int num, i;
num = (int)(slot - 'a');
/*if(slot_type[num]!='T')
{
//
send_online_framing(num,'T');
slot_type[num]='T';
}*/
for(i = 0; i < 4; i++)
{
if((parg[i] < 0x40) || (parg[i] > 0x57))
{
return 1;
}
}
memcpy(rpt_content.slot[num].rs_tzo, parg, 4);
return 0;
}
int save_ppx_mod(char slot, unsigned char *parg)
{
int num, i;
num = (int)(slot - 'a');
if(slot_type[num] != 'U')
{
//
send_online_framing(num, 'U');
slot_type[num] = 'U';
}
for(i = 0; i < 16; i++)
{
if((parg[i] != '1') && (parg[i] != '2') && (parg[i] != '3'))
{
return 1;
}
}
memcpy(rpt_content.slot[num].ppx_mod, parg, 16);
return 0;
}
int save_tod_en(char slot, unsigned char *parg)
{
int num, i;
num = (int)(slot - 'a');
if(slot_type[num] != 'V')
{
//
send_online_framing(num, 'V');
slot_type[num] = 'V';
}
for(i = 0; i < 16; i++)
{
if((parg[i] != '1') && (parg[i] != '0'))
{
return 1;
}
}
memcpy(rpt_content.slot[num].tod_en, parg, 16);
return 0;
}
int save_tod_pro(char slot, unsigned char *parg)
{
int num;
num = (int)(slot - 'a');
if(slot_type[num] != 'V')
{
//
send_online_framing(num, 'V');
slot_type[num] = 'V';
}
if((parg[0] < 0x30) || (parg[0] > 0x37))
{
return 1;
}
if((parg[2] < 0x40) || (parg[2] > 0x57))
{
return 1;
}
rpt_content.slot[num].tod_br = parg[0];
rpt_content.slot[num].tod_tzo = parg[2];
return 0;
}
int save_igb_en(char slot, unsigned char *parg)
{
int num, i;
num = (int)(slot - 'a');
if(slot_type[num] != 'W')
{
//
send_online_framing(num, 'W');
slot_type[num] = 'W';
}
for(i = 0; i < 4; i++)
{
if((parg[i] != '1') && (parg[i] != '0'))
{
return 1;
}
}
memcpy(rpt_content.slot[num].igb_en, parg, 4);
return 0;
}
int save_igb_rat(char slot, unsigned char *parg)
{
int num, i;
num = (int)(slot - 'a');
if(slot_type[num] != 'W')
{
//
send_online_framing(num, 'W');
slot_type[num] = 'W';
}
for(i = 0; i < 4; i++)
{
if((parg[i] < 'a') && (parg[i] > 'i'))
{
return 1;
}
}
memcpy(rpt_content.slot[num].igb_rat, parg, 4);
return 0;
}
int save_igb_tzone(char slot, unsigned char *parg)
{
int num;
num = (int)(slot - 'a');
if(slot_type[num] != 'W')
{
send_online_framing(num, 'W');
slot_type[num] = 'W';
}
rpt_content.slot[num].igb_tzone = parg[0];
return 0;
}
int save_igb_max(char slot, unsigned char *parg)
{
int num, i;
num = (int)(slot - 'a');
if(slot_type[num] != 'W')
{
//
send_online_framing(num, 'W');
slot_type[num] = 'W';
}
for(i = 0; i < 4; i++)
{
if((parg[i] < 'A') && (parg[i] > 'E'))
{
return 1;
}
}
memcpy(rpt_content.slot[num].igb_max, parg, 4);
return 0;
}
int check_net(char *parg,int len)
{
int i;
for(i=0;i<len;i++)
{
if( (parg[i]&0xf0) != 0x40)
{
return i;
}
else
{
}
}
return i;
}
int net_2_str(char h_4bit,char l_4bit)
{
if((h_4bit & 0xf0) != 0x40 || (l_4bit & 0xf0) != 0x40)
{
return -1;
}
else
{
return ( ((h_4bit&0x0f)<<4)|(l_4bit&0x0f) );
}
}
void __save_ntp_pnet(unsigned char *dit,char * parg,int len)
{
int i,res;
unsigned char str[6];
for(i=0;i<len;i+=2)
{
res = net_2_str(parg[i],parg[i+1]);
if(res >= 0)
{
str[i/2] = (unsigned char)res;
}
else
{
return;
}
}
memcpy(dit,str,len/2);
}
int save_ntp_pnet(char slot, unsigned char *parg)
{
int num, i,port,res;
int net_sta = 0;
int len;
unsigned char net_char[13];
num = (int)(slot - 'a');
len = strlen(parg);
port = 0;
for(i=0;i<len;i++)
{
memset(net_char,0,13);
if(parg[i]>='0' && parg[i]<='9')
{
net_sta = 0;
}
switch (net_sta)
{
case 0://port
//(parg[i+1] != ',')?(port = (parg[i++]-'0')*10+(parg[i++]-'0') ):(port = parg[i++]-'0' );
if(parg[i+1] != ',')
{
port = parg[i++]-'0';
port = port *10 + parg[i++]-'0';
}
else
{
port = parg[i++]-'0';
}
port -=1;
if(port < 0)
{
printf("port error\n");
net_sta = 5;
break;
}
net_sta = 1;
break;
case 1://mac
res = check_net(parg+i,len-i+1);
if(res == 12)
{
__save_ntp_pnet(rpt_content.slot[num].ntp_pmac[port],parg+i,res);
}
i = i+res;
net_sta = 2;
break;
case 2:
res = check_net(parg+i,len-i+1);
if(res == 8)
{
__save_ntp_pnet(rpt_content.slot[num].ntp_pip[port],parg+i,res);
}
i = i+res;
net_sta = 3;
break;
case 3:
res = check_net(parg+i,len-i+1);
if(res == 8)
{
__save_ntp_pnet(rpt_content.slot[num].ntp_pgateway[port],parg+i,res);
}
i = i+res;
net_sta = 4;
break;
case 4:
res = check_net(parg+i,len-i+1);
if(res == 8)
{
__save_ntp_pnet(rpt_content.slot[num].ntp_pnetmask[port],parg+i,res);
}
i = i+res;
net_sta = 1;
break;
case 5:
i = len+1;
break;
default:
break;
}
}
return 0;
}
int save_ntp_net(char slot, unsigned char *parg)
{
int num, i;
int j, i1, i2, i3, len;
j = i1 = i2 = i3 = 0;
num = (int)(slot - 'a');
len = strlen(parg);
if(slot_type[num] != 'Z')
{
//
send_online_framing(num, 'Z');
slot_type[num] = 'Z';
}
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
}
}
if(i3 == 0)
{
return 1;
}
if(parg[0] != ',')
{
memcpy(rpt_content.slot[num].ntp_ip, &parg[0], i1 - 1);
}
if(parg[i1] != ',')
{
memcpy(rpt_content.slot[num].ntp_mac, &parg[i1], i2 - i1 - 1);
}
if(parg[i2] != ',')
{
memcpy(rpt_content.slot[num].ntp_gate, &parg[i2], i3 - i2 - 1);
}
if(parg[i3] != '\0')
{
memcpy(rpt_content.slot[num].ntp_msk, &parg[i3], len - i3);
}
return 0;
}
int save_ntp_en(char slot, unsigned char *parg)
{
int num, i;
int j, i1, i2, i3, i4, len;
j = i1 = i2 = i3 = i4 = 0;
num = (int)(slot - 'a');
len = strlen(parg);
if(slot_type[num] != 'Z')
{
//
send_online_framing(num, 'Z');
slot_type[num] = 'Z';
}
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
}
}
if(i3 == 0)
{
return 1;
}
if((parg[0] == '0') || (parg[0] == '1'))
{
rpt_content.slot[num].ntp_bcast_en = parg[0];
}
if(parg[i1] != ',')
{
memset(rpt_content.slot[num].ntp_interval, 0, sizeof(rpt_content.slot[num].ntp_interval));
memcpy(rpt_content.slot[num].ntp_interval, &parg[i1], i2 - i1 - 1);
}
if((parg[i2] > 0x3f) && (parg[i2] < 0x48))
{
rpt_content.slot[num].ntp_tpv_en = parg[i2];
}
if((parg[i3] == '0') || (parg[i3] == '1'))
{
rpt_content.slot[num].ntp_md5_en = parg[i3];
}
/*
if((parg[i4] > 0x3f) && (parg[i4] < 0x48))
{
rpt_content.slot[num].ntp_ois_en = parg[i4];
}
*/
return 0;
}
int save_r_ntp_key(char slot, unsigned char *parg)
{
int num, i;
int len, i1, i2, i3, i4, i5, i6, i7, j;
len = i1 = i2 = i3 = i4 = i5 = i6 = i7 = j = 0;
num = (int)(slot - 'a');
len = strlen(parg);
if(slot_type[num] != 'Z')
{
//
send_online_framing(num, 'Z');
slot_type[num] = 'Z';
}
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
if(j == 5)
{
i5 = i + 1;
}
if(j == 6)
{
i6 = i + 1;
}
if(j == 7)
{
i7 = i + 1;
}
}
}
if(i7 == 0)
{
return 1;
}
memset(rpt_content.slot[num].ntp_keyid, '\0', 20);
memset(rpt_content.slot[num].ntp_key, '\0', 68);
if((parg[0] != ',') && (parg[i1] != ','))
{
memcpy(rpt_content.slot[num].ntp_keyid[0], &parg[0], i1 - 1);
memcpy(rpt_content.slot[num].ntp_key[0], &parg[i1], i2 - i1 - 1);
}
if((parg[i2] != ',') && (parg[i3] != ','))
{
memcpy(rpt_content.slot[num].ntp_keyid[1], &parg[i2], i3 - i2 - 1);
memcpy(rpt_content.slot[num].ntp_key[1], &parg[i3], i4 - i3 - 1);
}
if((parg[i4] != ',') && (parg[i5] != ','))
{
memcpy(rpt_content.slot[num].ntp_keyid[2], &parg[i4], i5 - i4 - 1);
memcpy(rpt_content.slot[num].ntp_key[2], &parg[i5], i6 - i5 - 1);
}
if((parg[i6] != ',') && (parg[i7] != '\0'))
{
memcpy(rpt_content.slot[num].ntp_keyid[3], &parg[i6], i7 - i6 - 1);
memcpy(rpt_content.slot[num].ntp_key[3], &parg[i7], len - i7);
}
return 0;
}
int save_ntp_key(char slot, unsigned char *parg)
{
int num, i;
unsigned char keyid[5];
memset(keyid, '\0', 5);
num = (int)(slot - 'a');
if(slot_type[num] != 'Z')
{
//
send_online_framing(num, 'Z');
slot_type[num] = 'Z';
}
if((parg[0] != '0') && (parg[0] != '1') && (parg[0] != '2'))
{
return 1;
}
else if(parg[1] != ',')
{
return 1;
}
else if(parg[6] != ',')
{
return 1;
}
memcpy(keyid, &parg[2], 4);
if(parg[0] == '0')
{
for(i = 0; i < 4; i++)
{
//if(rpt_content.slot[num].ntp_key_flag[i]!=2)
if(strncmp1(rpt_content.slot[num].ntp_keyid[i], keyid, 4) == 0)
{
return 1;
}
else if(rpt_content.slot[num].ntp_keyid[i][0] == '\0')
{
// rpt_content.slot[num].ntp_key_flag[i]=2;
memcpy(rpt_content.slot[num].ntp_keyid[i], &parg[2], 4);
memcpy(rpt_content.slot[num].ntp_key[i], &parg[7], 16);
break;
}
else if(i == 3)
{
return 1;
}
}
}
else if(parg[0] == '1')
{
for(i = 0; i < 4; i++)
{
if(strncmp1(keyid, rpt_content.slot[num].ntp_keyid[i] , 4) == 0)
{
//rpt_content.slot[num].ntp_key_flag[i]=1;
memset(rpt_content.slot[num].ntp_keyid[i], '\0', 5);
memset(rpt_content.slot[num].ntp_key[i], '\0', 17);
break;
}
else if(i == 3)
{
return 1;
}
}
}
else if(parg[0] == '2')
{
for(i = 0; i < 4; i++)
{
if(strncmp1(keyid, rpt_content.slot[num].ntp_keyid[i] , 4) == 0)
{
memset(rpt_content.slot[num].ntp_key[i], '\0', 17);
memcpy(rpt_content.slot[num].ntp_key[i], &parg[7], 16);
break;
}
else if(i == 3)
{
return 1;
}
}
}
return 0;
}
int save_ntp_ms(char slot, unsigned char *parg)
{
int num, len;
num = (int)(slot - 'a');
len = strlen(parg);
if(slot_type[num] != 'Z')
{
//
send_online_framing(num, 'Z');
slot_type[num] = 'Z';
}
if((parg[0] != '0') && (parg[0] != '1'))
{
return 1;
}
if(len < 4)
{
return 1;
}
memcpy(rpt_content.slot[num].ntp_ms, parg, len);
return 0;
}
int save_ntp_sta(char slot, unsigned char *parg)
{
int num, i;
int j, i1, i2, i3, len;
j = i1 = i2 = i3 = 0;
num = (int)(slot - 'a');
len = strlen(parg);
if(0 == len)
{
return 1;
}
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
}
}
if(i1 == 0)
{
memset(rpt_content.slot[num].ntp_leap,0,sizeof(rpt_content.slot[num].ntp_leap));
memcpy(rpt_content.slot[num].ntp_leap, &parg[0], len);
}
else
{
if(parg[0] != ',')
{
memset(rpt_content.slot[num].ntp_leap,0,sizeof(rpt_content.slot[num].ntp_leap));
memcpy(rpt_content.slot[num].ntp_leap, &parg[0], i1 - 1);
}
if(parg[i1] != '\0')
{
memset(rpt_content.slot[num].ntp_mcu,0,sizeof(rpt_content.slot[num].ntp_mcu));
memcpy(rpt_content.slot[num].ntp_mcu, &parg[i1], len - i1);
}
}
return 0;
}
int save_ntp_psta(char slot, unsigned char *parg)
{
int num;
num = (int)(slot - 'a');
if(slot_type[num] != 'Z')
{
send_online_framing(num, 'Z');
slot_type[num] = 'Z';
}
memset(rpt_content.slot[num].ntp_portEn,0,sizeof(rpt_content.slot[num].ntp_portEn));
memset(rpt_content.slot[num].ntp_portStatus,0,sizeof(rpt_content.slot[num].ntp_portStatus));
rpt_content.slot[num].ntp_portEn[0] = parg[0];
rpt_content.slot[num].ntp_portEn[1] = parg[1];
rpt_content.slot[num].ntp_portEn[2] = parg[2];
rpt_content.slot[num].ntp_portEn[3] = parg[3];
rpt_content.slot[num].ntp_portStatus[0] = parg[5];
rpt_content.slot[num].ntp_portStatus[1] = parg[6];
rpt_content.slot[num].ntp_portStatus[2] = parg[7];
rpt_content.slot[num].ntp_portStatus[3] = parg[8];
return 0;
}
int save_ntp_alm(char slot, unsigned char *parg)
{
int num;
unsigned char tmp[14];
memset(tmp, '\0', 14);
rpt_alm_to_client(slot, parg);
num = (int)(slot - 'a');
sprintf((char *)tmp, "\"%c,Z,%s\"", slot, parg);
memcpy(alm_sta[num], tmp, strlen(tmp));
return 0;
}
int save_2mb_lvlm(char slot, unsigned char *parg)
{
int num;
num = (int)(slot - 'a');
/*if(slot_type[num]!='T')
{
//
send_online_framing(num,'T');
slot_type[num]='T';
}*/
//for(i = 0; i < 4; i++)
//{
// if((parg[i] < 0x40) || (parg[i] > 0x57))
// {
if((parg[0] != '1')&&(parg[0] != '0'))
return 1;
// }
//}
rpt_content.slot[num].ref_2mb_lvlm = parg[0];
return 0;
}
int save_rb_sa(char slot, unsigned char *parg)
{
if((parg[0] < '@') || (parg[0] > 'E'))
{
return 1;
}
if(slot == RB1_SLOT)
{
rpt_content.slot_o.rb_sa = parg[0];
}
else if(slot == RB2_SLOT)
{
rpt_content.slot_p.rb_sa = parg[0];
}
return 0;
}
int save_ok(char slot, unsigned char *parg)
{
return 0;
}
/*
璁剧疆闂扮锛?
鎴愬姛0锛?
澶辫触1锛?
*/
/*
struct StrTime
{
unsigned int hour;
unsigned int minute;
unsigned int second;
};
struct StrDate
{
unsigned int year;
unsigned int month;
unsigned int day;
};
struct StrTime msgTime;
struct StrDate msgDate;
struct StrTime mcpTime;
struct StrDate mcpDate;
int getLeapDate(char * msg)
{
int i=0;
int len=0;
int cnt = 0;
char tmp[11];
get_time_fromnet();
while(mcp_date[i]!='\0' && i<11)
{
if('-' == mcp_date[i])
{
memset(tmp,0,11);
if(cnt == 0)
{
cnt ++;
memcpy(tmp,&mcp_date[i-4],4);
mcpDate.year = atoi(tmp);
}
else if(cnt == 1)
{
cnt ++;
memcpy(tmp,&mcp_date[i-2],2);
mcpDate.month= atoi(tmp);
}
else if(cnt == 2)
{
cnt ++;
memcpy(tmp,&mcp_date[i-2],2);
mcpDate.day= atoi(tmp);
break;
}
else
{
printf("%s mcp date error\n",__FUNCTION__);
return 0;
}
}
i++;
}
i=0;
cnt = 0;
while(mcp_time[i]!='\0' && i<11)
{
if(':' == mcp_time[i])
{
memset(tmp,0,11);
if(cnt == 0)
{
cnt ++;
memcpy(tmp,&mcp_time[i-2],2);
mcpTime.hour = atoi(tmp);
}
else if(cnt == 1)
{
cnt ++;
memcpy(tmp,&mcp_time[i-2],2);
mcpTime.minute= atoi(tmp);
}
else if( 2 == cnt )
{
cnt ++;
memcpy(tmp,&mcp_time[i-2],2);
mcpTime.second= atoi(tmp);
break;
}
else
{
//printf("%s mcp date error\n",__FUNCTION__);
//return 0;
}
}
i++;
}
//L,235960,31,12,2012,15,16,01;
i = 0;
cnt = 0;
while(msg[i] != '\0' && i < len)
{
if(',' == msg[i])
{
memset(tmp,0,11);
if( cnt == 0)
{
cnt ++;
}
else if( cnt == 1)
{
cnt++;
memset(tmp,0,11);
memcpy(tmp,&msg[i-6],2);
msgTime.hour = atoi(tmp);
memset(tmp,0,11);
memcpy(tmp,&msg[i-4],2);
msgTime.minute= atoi(tmp);
memset(tmp,0,11);
memcpy(tmp,&msg[i-2],2);
msgTime.second= atoi(tmp);
}
else if( 2 == cnt)
{
cnt ++;
memset(tmp,0,11);
memcpy(tmp,&msg[i-2],2);
msgDate.day= atoi(tmp);
}
else if( 3 == cnt )
{
cnt ++;
memset(tmp,0,11);
memcpy(tmp,&msg[i-2],2);
msgDate.month= atoi(tmp);
}
else if( 4 == cnt)
{
cnt ++;
memset(tmp,0,11);
memcpy(tmp,&msg[i-4],4);
msgDate.year= atoi(tmp);
}
else
{
printf("leap msg error \n");
return 1;
}
}
}
}
*/
int save_leap_alm(char slot, unsigned char *parg)
{
int i;
int cnt = 0;
int len;
char msg[64];
char tmp[11];
char leapTime[20];
char leapDate[20];
len = strlen(parg);
if(len > 64)
return 1;
memset(msg, 0, 64);
memcpy(msg, parg, len);
printf("@#%s\n", __FUNCTION__);
if(g_LeapFlag != 0)
{
return 1;
}
else
{
;
}
for(i = 0; i < len; i++)
{
if(',' == msg[i])
{
cnt++;
}
else
{
continue;
}
}
if(cnt != 6)
{
return 1;
}
else
{
memset(tmp, 0, 11);
memset(leapDate, 0, 20);
memset(leapTime, 0, 20);
i = 0;
cnt = 0;
}
while(msg[i] != '\0' && i < len)
{
if(',' == msg[i])
{
memset(tmp, 0, 11);
if( cnt == 0)
{
cnt++;
memset(tmp, 0, 11);
memcpy(tmp, &msg[i - 6], 2);
sprintf((char *)leapTime, "%s", tmp);
memset(tmp, 0, 11);
memcpy(tmp, &msg[i - 4], 2);
sprintf((char *)leapTime, "%s:%s", leapTime, tmp);
memset(tmp, 0, 11);
memcpy(tmp, &msg[i - 2], 2);
sprintf((char *)leapTime, "%s:%s", leapTime, tmp);
}
else if( 1 == cnt)
{
cnt ++;
memset(tmp, 0, 11);
memcpy(tmp, &msg[i - 2], 2);
sprintf((char *)leapDate, "%s", tmp);
}
else if( 2 == cnt )
{
cnt ++;
memset(tmp, 0, 11);
memcpy(tmp, &msg[i - 2], 2);
sprintf((char *)tmp, "%s-%s", tmp, leapDate);
memset(leapDate , 0, 20);
memcpy(leapDate, tmp, strlen(tmp));
}
else if( 3 == cnt)
{
cnt ++;
memset(tmp, 0, 11);
memcpy(tmp, &msg[i - 4], 4);
sprintf((char *)tmp, "%s-%s", tmp, leapDate);
memset(leapDate , 0, 20);
memcpy(leapDate, tmp, strlen(tmp));
}
else if(5 == cnt)
{
cnt++;
//R-LEAP-ALM :L,235960,31,12,2012,15,16,01;
;
}
else if(4 == cnt)
{
cnt++;
memset(tmp, 0, 11);
memcpy(tmp, &msg[i - 2], 2);
if( (tmp[0] >= '0' && tmp[0] <= '9') &&
(tmp[1] >= '0' && tmp[1] <= '9') )
{
memset(conf_content.slot_u.leap_num, '\0', 3);
memcpy(conf_content.slot_u.leap_num, tmp, 2);
}
else
{
return 1;
}
}
}
i++;
}
if((msg[len-2] == '0')&&((msg[len-1] == '0')||(msg[len-1] == '1')||(msg[len-1] == '2')))
{
rpt_content.slot_q.leapalm[0] = msg[len-2];
rpt_content.slot_q.leapalm[1] = msg[len-1];
rpt_content.slot_q.leapalm[2] = '\0';
}
else
{
rpt_content.slot_q.leapalm[0] = '0';
rpt_content.slot_q.leapalm[1] = '0';
rpt_content.slot_q.leapalm[2] = '\0';
}
#if 0
get_time_fromnet();
ret = strncmp1( mcp_date, leapDate, strlen(mcp_date));
if( ret <= 0)
{
g_LeapFlag = 1;
}
else
{
g_LeapFlag = 0;
return 1;
}
ret = strncmp1( mcp_time, leapTime, strlen(mcp_time));
if( ret <= 0)
{
g_LeapFlag = 1;
}
else
{
g_LeapFlag = 0;
return 1;
}
#endif
g_LeapFlag = 1;
printf("###%s\n", conf_content.slot_u.leap_num);
return 0;
}
void sta1_alm(unsigned char *sendbuf, unsigned char slot)
{
if( 1 == (alm_sta[20][1] & 0x01) )
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/GGIO1/Alm=1;");
sendtodown(sendbuf, slot);
}
if( 0x02 == (alm_sta[20][1] & 0x02))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/GGIO1/Alm1=1;");
sendtodown(sendbuf, slot);
}
}
void sta1_portnum(unsigned char *sendbuf, unsigned char slot)
{
unsigned char i;
unsigned char portNum = 0;
for(i = 0; i < 14; i++)
{
if( 'O' != slot_type[i] )
{
portNum++;
}
}
//memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/LLN0/PortNum=%d;", portNum);
sendtodown(sendbuf, slot);
}
void sta1_net(unsigned char *sendbuf, unsigned char slot)
{
#if 1
unsigned char net_buf[50];
unsigned int net_ip;
int s;
struct ifreq ifr;
struct sockaddr_in *ptr;
unsigned char *interface_name = "eth0";
if((s = socket(AF_INET, SOCK_STREAM, 0)) < 0)
{
perror("Socket");
return;
}
strncpy(ifr.ifr_name, interface_name, IFNAMSIZ);
if(ioctl(s, SIOCGIFADDR, &ifr) < 0)
{
perror("Get_McuLocalIp ioctl");
close(s);
}
ptr = (struct sockaddr_in *)&ifr.ifr_ifru.ifru_addr;
net_ip = (unsigned int)(ptr->sin_addr.s_addr);
//strcpy(mcp_local_ip,(unsigned char *)inet_ntoa (ptr->sin_addr));
close(s);
//IPAddr
//memset(net_buf,0,50);
//API_Get_McpIp(net_buf);
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/LLN0/IPAddr=%x;", net_ip);
sendtodown(sendbuf, slot);
//MACAddr
memset(net_buf, 0, 50);
memset(sendbuf, '\0', SENDBUFSIZE);
API_Get_McpMacAddr(net_buf);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/LLN0/MACAddr=%s;", net_buf);
sendtodown(sendbuf, slot);
#endif
}
void sta1_cpu(unsigned char *sendbuf, unsigned char slot)
{
//CpuUsage
//memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/LLN0/CpuUsage=67;");
sendtodown(sendbuf, slot);
}
void sta1_in_out(unsigned char *sendbuf, unsigned char slot)
{
unsigned char temp = 0;
unsigned char i = 0;
//00010001
//GPS BD
if('O' != slot_type[16])
{
if( (strncmp(rpt_content.slot_q.gb_acmode, "BD", 2) == 0) )
{
temp |= 0x01;
}
else
{
temp |= 0x10;
}
}
if('O' != slot_type[17] )
{
if( (strncmp(rpt_content.slot_r.gb_acmode, "BD", 2) == 0) )
{
temp |= 0x01;
}
else
{
temp |= 0x10;
}
}
//memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/LLN0/InType=");
if((temp & 0x10) == 0x10)
{
sprintf((char *)sendbuf, "%sGPS ", sendbuf);
}
if((temp & 0x01) == 0x01)
{
sprintf((char *)sendbuf, "%sBD ", sendbuf);
}
temp = strlen(sendbuf);
if(sendbuf[temp - 1] == ' ')
{
sendbuf[temp - 1] = ';';
}
if(sendbuf[temp - 1] == ';')
{
sendtodown(sendbuf, slot);
}
temp = 0;
//111000
//b鐮?588 pps+tod
for(i = 0; i < 14; i++)
{
if('W' == slot_type[i])
{
temp |= 0x80;
}
if( (slot_type[i] >= 'A' && slot_type[i] <= 'H')
|| (slot_type[i] >= 'n' && slot_type[i] <= 'q') )
{
temp |= 0x40;
}
if( 'I' == slot_type[i] )
{
temp |= 0x20;
}
}
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/LLN0/OutType=");
if( (temp & 0x80) == 0x80)
{
sprintf((char *)sendbuf, "%sB鐮?", sendbuf);
}
if( (temp & 0x40) == 0x40 )
{
sprintf((char *)sendbuf, "%s1588 ", sendbuf);
}
if( (temp & 0x20) == 0x20 )
{
sprintf((char *)sendbuf, "%s1PPS+TOD ", sendbuf);
}
temp = strlen(sendbuf);
if(sendbuf[temp - 1] == ' ')
{
sendbuf[temp - 1] = ';';
}
if(sendbuf[temp - 1] == ';')
{
sendtodown(sendbuf, slot);
}
}
void send_61850_sta(unsigned char slot)
{
unsigned char sendbuf[125];
sta1_alm(sendbuf, slot);
sta1_portnum(sendbuf, slot);
sta1_net(sendbuf, slot);
sta1_cpu(sendbuf, slot);
sta1_in_out(sendbuf, slot);
}
int save_sta1_alm(char slot, unsigned char *parg)
{
int num;
unsigned char tmp[14];
if(slot < 'a' || slot > 'n')
{
return -1;
}
else
{
num = (int)(slot - 'a');
}
memset(tmp, '\0', 14);
rpt_alm_to_client(slot, parg);
sprintf((char *)tmp, "\"%c,m,%s\"", slot, parg);
memcpy(alm_sta[num], tmp, strlen(tmp));
send_61850_sta(slot);
return 0;
}
//
//!PGEIN单盘输入100S上报保存网络参数/事件CD1
//
int save_pgein_net(char slot, unsigned char *parg)
{
int num, port;
int i, j, i1, i2, i3,len;
j = i1 = i2 = i3 = 0;
num = (int)(slot - 'a');
port = (int)(parg[0] - 0x31);
len = strlen(parg);
//
//!PI NET PRINTF .
//
//printf("PI NET %s\n",parg);
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
}
}
if(i3 == 0)
{
return (1);
}
//
//! ip address
//
if(parg[i1] != ',')
{
memcpy(rpt_content.slot[num].ptp_ip[port], &parg[i1], i2 - i1 - 1);
}
//
//! gate address
//
if(parg[i2] != ',')
{
memcpy(rpt_content.slot[num].ptp_gate[port], &parg[i2], i3 - i2 - 1);
}
//
//! mask address
//
if(parg[i3] != '\0')
{
memcpy(rpt_content.slot[num].ptp_mask[port], &parg[i3], len - i3);
}
#if DEBUG_NET_INFO
if(print_switch == 0)
printf("PI NET %s:%s,%s,%s#\n", NET_SAVE, rpt_content.slot[num].ptp_ip[port],
rpt_content.slot[num].ptp_gate[port],
rpt_content.slot[num].ptp_mask[port]);
#endif
return 0;
}
//
//! PGEIN 单盘输入 工作模式100S上报/事件CD2
//
int save_pgein_mod(char slot, unsigned char *parg)
{
int i, j, i1, i2, i3, i4, i5, i6, len;
int num, port;
j = i1 = i2 = i3 = i4 = i5 = i6 = 0;
num = (int)(slot - 'a');
port = (int)(parg[0] - 0x31);
len = strlen(parg);
//
//! PGEIN
//
//printf("PI MOD %s\n",parg);
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
if(j == 5)
{
i5 = i + 1;
}
if(j == 6)
{
i6 = i + 1;
}
}
}
if(i6 == 0)
{
return (1);
}
//
//! E2E/P2P
//
if((parg[i1] == '1') || (parg[i1] == '0'))
{
rpt_content.slot[num].ptp_delaytype[port] = parg[i1];
rpt_content.slot[num].ptp_delaytype[1] = '\0';
//
//!PGEIN
//
//printf("PI Delaytype %d,%c\n",port,rpt_content.slot[num].ptp_delaytype[port]);
}
//
//! multicast /uniticast
//
if((parg[i2] == '1') || (parg[i2] == '0'))
{
rpt_content.slot[num].ptp_multicast[port] = parg[i2];
rpt_content.slot[num].ptp_multicast[1] = '\0';
}
//
//!enq two /three
//
if((parg[i3] == '1') || (parg[i3] == '0'))
{
rpt_content.slot[num].ptp_enp[port] = parg[i3];
rpt_content.slot[num].ptp_enp[1] = '\0';
}
//
//! one step/two step
//
if((parg[i4] == '1') || (parg[i4] == '0'))
{
rpt_content.slot[num].ptp_step[port] = parg[i4];
rpt_content.slot[num].ptp_step[1] = '\0';
}
//
//!delay req fre
//
if(parg[i5] != ',')
{
memcpy(rpt_content.slot[num].ptp_delayreq[port], &parg[i5], i6 - i5 - 1);
rpt_content.slot[num].ptp_delayreq[port][2] = '\0';
}
//
//!pdelay req fre
//
if(parg[i6] != '\0')
{
memcpy(rpt_content.slot[num].ptp_pdelayreq[port], &parg[i6], len - i6);
rpt_content.slot[num].ptp_pdelayreq[port][2] = '\0';
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("PI MOD %s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
//
//!PGEIN单盘输入参考输入信号质量变化事件CD3
//
int save_pgein_clkclass(char slot, unsigned char *parg)
{
int num, port;
int i, j, i1,len;
j = i1 = 0;
num = (int)(slot - 'a');
port = (int)(parg[0] - 0x31);
len = strlen(parg);
//
//! clock class
//
//printf("PI clkcls %s\n",parg);
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
}
}
if(i1 == 0)
{
return (1);
}
//
//! clkclass
//
if(parg[i1] != '\0')
{
memcpy(rpt_content.slot[num].ptp_clkcls[port], &parg[i1], len - i1);
rpt_content.slot[num].ptp_clkcls[port][3] = '\0';
}
#if DEBUG_NET_INFO
if(print_switch == 0)
printf("PI CLK %s:%s#\n", NET_SAVE, rpt_content.slot[num].ptp_clkcls[port]);
#endif
return 0;
}
//
//! 100S上报报警
//
int save_pgein_alm(char slot, unsigned char *parg)
{
int num;
unsigned char tmp[14];
memset(tmp, '\0', 14);
rpt_alm_to_client(slot, parg);
num = (int)(slot - 'a');
sprintf((char *)tmp, "\"%c,%c,%s\"", slot, slot_type[num], parg);
memcpy(alm_sta[num], tmp, strlen(tmp));
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("PGEIN %s:%s#\n", NET_SAVE, parg);
}
#endif
return 0;
}
//
//!PGE4V2单盘输出100S上报
//
int save_pge4v_net(char slot, unsigned char *parg)
{
int num, port;
int i, j, i1, i2, i3, i4,len;
unsigned char datalen;
unsigned char errflag;
datalen = errflag = 0;
j = i1 = i2 = i3 = i4 = 0;
num = (int)(slot - 'a');
port = (int)(parg[0] - 0x31);
len = strlen(parg);
//
//slot range 0 ~ 13。
//
if((num < 0) || (num > 13))
return 1;
//
//port range 0 ~ 3。
//
if((port < 0) ||(port > 3))
return 1;
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
}
}
//
//error ,return 1。
if(i4 == 0)
{
return (1);
}
//
//Judge the data len or not correct.
//
datalen = i2 - i1 -1;
if(datalen > MAX_MAC_LEN)
errflag = 1;
datalen = i3 - i2 -1;
if(datalen > MAX_IP_LEN)
errflag = 1;
datalen = i4 - i3 -1;
if(datalen > MAX_IP_LEN)
errflag = 1;
datalen = len - i4;
if(datalen > MAX_IP_LEN)
errflag = 1;
if(1 == errflag)
return 1;
//
//! mac address
//
if(parg[i1] != ',')
{
memset(rpt_content.slot[num].ptp_mac[port],'\0',18);
memcpy(rpt_content.slot[num].ptp_mac[port], &parg[i1], i2 - i1 - 1);
}
//
//! ip address
//
if(parg[i2] != ',')
{
memset(rpt_content.slot[num].ptp_ip[port],'\0',MAX_IP_LEN + 1);
memcpy(rpt_content.slot[num].ptp_ip[port], &parg[i2], i3 - i2 - 1);
}
//
//! mask address
//
if(parg[i3] != ',')
{
memset(rpt_content.slot[num].ptp_mask[port],'\0',MAX_IP_LEN + 1);
memcpy(rpt_content.slot[num].ptp_mask[port], &parg[i3], i4 - i3 - 1);
}
//
//! gate address
//
if(parg[i4] != '\0')
{
memset(rpt_content.slot[num].ptp_gate[port],'\0',MAX_IP_LEN + 1);
memcpy(rpt_content.slot[num].ptp_gate[port], &parg[i4], len - i4);
}
#if DEBUG_NET_INFO
if(print_switch == 0)
printf("PGE4S NET %s:%s,%s,%s,%s#\n", NET_SAVE, rpt_content.slot[num].ptp_mac[port],
rpt_content.slot[num].ptp_ip[port],
rpt_content.slot[num].ptp_gate[port],
rpt_content.slot[num].ptp_mask[port]);
#endif
return 0;
}
//
//!PGE4S单盘输出工作模式100S上报
//
int save_pge4v_mod(char slot, unsigned char *parg)
{
int num, port;
int i, j, i1, i2, i3, i4, i5, i6, i7, i8, i9, i10, i11, i12, i13,len;
unsigned char datalen;
datalen = 0;
j = i1 = i2 = i3 = i4 = i5 = i6 = 0;
i7 = i8 = i9 = i10 = i11 = i12 = i13 = 0;
//
//num , port , len ;
num = (int)(slot - 'a');
port = (int)(parg[0] - 0x31);
len = strlen(parg);
//
//slot range 0 ~ 13。
//
if((num < 0) || (num > 13))
return 1;
//
//port range 0 ~ 3。
//
if((port < 0) ||(port > 3))
return 1;
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
if(j == 5)
{
i5 = i + 1;
}
if(j == 6)
{
i6 = i + 1;
}
if(j == 7)
{
i7 = i + 1;
}
if(j == 8)
{
i8 = i + 1;
}
if(j == 9)
{
i9 = i + 1;
}
if(j == 10)
{
i10 = i + 1;
}
if(j == 11)
{
i11 = i + 1;
}
if(j == 12)
{
i12 = i + 1;
}
if(j == 13)
{
i13 = i + 1;
}
}
}
//
//error ,return 1。
if(i12 == 0)
{
return (1);
}
//
//! enable。
//
if((parg[i1] == '0') || (parg[i1] == '1'))
{
rpt_content.slot[num].ptp_en[port] = parg[i1];
}
//
//! esmc enable。
//
if((parg[i2] == '0') || (parg[i2] == '1'))
{
rpt_ptp_mtc.esmc_en[num][port] = parg[i2];
}
//
//! delaytype
//
if((parg[i3] == '0') || (parg[i3] == '1'))
{
rpt_content.slot[num].ptp_delaytype[port] = parg[i3];
}
//
//! multicast
//
if((parg[i4] == '0') || (parg[i4] == '1'))
{
rpt_content.slot[num].ptp_multicast[port] = parg[i4];
}
//
//! enp
//
if((parg[i5] == '0') || (parg[i5] == '1'))
{
rpt_content.slot[num].ptp_enp[port] = parg[i5];
}
//
//! step
//
if((parg[i6] == '0') || (parg[i6] == '1'))
{
rpt_content.slot[num].ptp_step[port] = parg[i6];
}
//
//! domain
//
if(parg[i7] != ',')
{
datalen = i8 - i7 - 1;
if(datalen <= MAX_DOM_LEN)
{
memset(rpt_ptp_mtc.ptp_dom[num][port],'\0', 4);
memcpy(rpt_ptp_mtc.ptp_dom[num][port], &parg[i7], datalen);
}
}
//
//!sync fre
//
if(parg[i8] != ',')
{
datalen = i9 - i8 - 1;
if(datalen == FRAME_FRE_LEN)
memcpy(rpt_content.slot[num].ptp_sync[port], &parg[i8], datalen);
}
//
//!announce。
//
if(parg[i9] != ',')
{
datalen = i10 - i9 - 1;
if(datalen == FRAME_FRE_LEN)
memcpy(rpt_content.slot[num].ptp_announce[port], &parg[i9], datalen);
}
//
//!delaycom
//
if(parg[i10] != ',')
{
datalen = i11 - i10 - 1;
if(datalen <= DELAY_COM_LEN)
{
memset(rpt_content.slot[num].ptp_delaycom[port],'\0', 9);
memcpy(rpt_content.slot[num].ptp_delaycom[port], &parg[i10], datalen);
}
}
//
//!prio 1
//
if(parg[i11] != ',')
{
datalen = i12 - i11 - 1;
if(datalen <= MAX_PRIO_LEN)
{
memset(rpt_ptp_mtc.ptp_prio1[num][port],'\0', 5);
memcpy(rpt_ptp_mtc.ptp_prio1[num][port], &parg[i11], datalen);
}
}
//
//! prio 2
//
if(parg[i12] != ',')
{
datalen = i13 - i12 - 1;
if(datalen <= MAX_PRIO_LEN)
{
memset(rpt_ptp_mtc.ptp_prio2[num][port],'\0', 5);
memcpy(rpt_ptp_mtc.ptp_prio2[num][port], &parg[i12], datalen);
}
}
//
//! ptp versionptp_ver
//
if(parg[i13] != '\0')
{
datalen = len - i13;
if(datalen <= 9)
{
memset(rpt_ptp_mtc.ptp_ver[num][port],'\0', 10);
memcpy(rpt_ptp_mtc.ptp_ver[num][port], &parg[i13], datalen);
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
printf("PGE4S MOD %s:%s#\n", NET_SAVE, rpt_ptp_mtc.ptp_ver[num][port]);
#endif
return 0;
}
//
//!PGE4S单盘输出单播对端100S上报
//
int save_pge4v_mtc(char slot, unsigned char *parg)
{
int num, port;
int i, j, i1, i2, i3, i4,len;//, i5, i6, i7, i8, i9, i10, i11, i12, i13
unsigned char datalen;
datalen = 0;
j = i1 = i2 = i3 =i4 = 0;
//
//num , port , len ;
//
num = (int)(slot - 'a');
port = (int)(parg[0] - 0x31);
len = strlen(parg);
//
//slot range 0 ~ 13。
//
if((num < 0) || (num > 13))
return 1;
//
//port range 0 ~ 3。
//
if((port < 0) ||(port > 3))
return 1;
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
}
}
//
//error ,return 1。
if(i4 == 0)
{
return (1);
}
//
//! mtc ip。
//
if(parg[i1] != ',')
{
datalen = i2 - i1 - 1;
if(datalen <= MAX_IP_LEN)
{
memset(rpt_ptp_mtc.ptp_mtc_ip[num][port], '\0', 9);
memcpy(rpt_ptp_mtc.ptp_mtc_ip[num][port], &parg[i1], datalen);
}
}
//
//! sfp modul type。
//
if(parg[i2] != ',')
{
datalen = i3 - i2 - 1;
if(datalen == FRAME_FRE_LEN)
{
memset(rpt_ptp_mtc.sfp_type[num][port], '\0', 3);
memcpy(rpt_ptp_mtc.sfp_type[num][port], &parg[i2], datalen);
}
}
//
//! op low.
//
if(parg[i3] != ',')
{
datalen = i4 - i3 - 1;
if(datalen <= MAX_OPTHR_LEN)
{
memset(rpt_ptp_mtc.sfp_oplo[num][port], '\0', 7);
memcpy(rpt_ptp_mtc.sfp_oplo[num][port], &parg[i3], datalen);
}
}
//
//! op hi.
//
if(parg[i4] != '\0')
{
datalen = len - i4;
if(datalen <= MAX_OPTHR_LEN)
{
memset(rpt_ptp_mtc.sfp_ophi[num][port], '\0', 7);
memcpy(rpt_ptp_mtc.sfp_ophi[num][port], &parg[i4], datalen);
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
printf("PGE4S%s:%s#\n", NET_SAVE, rpt_ptp_mtc.ptp_mtc_ip[num][port]);
#endif
return 0;
}
//
//!PGE4S单盘输出PTP状态字节100S上报
//
int save_pge4v_sta(char slot, unsigned char *parg)
{
int num, port;
int i, j, i1, i2, i3, i4,len;//, i5, i6, i7, i8, i9, i10, i11, i12, i13
unsigned char datalen;
datalen = 0;
j = i1 = i2 = i3 =i4 = 0;
//
//num , port , len ;
//
num = (int)(slot - 'a');
port = (int)(parg[0] - 0x31);
len = strlen(parg);
//
//slot range 0 ~ 13。
//
if((num < 0) || (num > 13))
return 1;
//
//port range 0 ~ 3。
//
if((port < 0) ||(port > 3))
return 1;
for(i = 0; i < len; i++)
{
if(parg[i] == ',')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
}
}
//
//error ,return 1。
if(i4 == 0)
{
return (1);
}
//
//! time source。
//
if(parg[i1] != ',')
{
datalen = i2 - i1 - 1;
if(datalen == MAX_TIMES_LEN)
{
memset(rpt_ptp_mtc.time_source[num][port], '\0', 5);
memcpy(rpt_ptp_mtc.time_source[num][port], &parg[i1], datalen);
}
}
//
//! clock class。
//
if(parg[i2] != ',')
{
datalen = i3 - i2 - 1;
if(datalen <= MAX_CLKCLS_LEN)
{
memset(rpt_ptp_mtc.clock_class[num][port], '\0', 4);
memcpy(rpt_ptp_mtc.clock_class[num][port], &parg[i2], datalen);
}
}
//
//! time acc.
//
if(parg[i3] != ',')
{
datalen = i4 - i3 - 1;
if(datalen <= MAX_TIMES_LEN)
{
memset(rpt_ptp_mtc.time_acc[num][port], '\0', 5);
memcpy(rpt_ptp_mtc.time_acc[num][port], &parg[i3], datalen);
}
}
//
//! synce ssm.
//
if(parg[i4] != '\0')
{
datalen = len - i4;
if(datalen <= MAX_SYNCSSM_LEN)
{
memset(rpt_ptp_mtc.synce_ssm[num][port], '\0', 14);
memcpy(rpt_ptp_mtc.synce_ssm[num][port], &parg[i4], datalen);
}
}
#if DEBUG_NET_INFO
if(print_switch == 0)
printf("PGE4S%s:%s#\n", NET_SAVE, rpt_ptp_mtc.synce_ssm[num][port]);
#endif
return 0;
}
//
//!PGE4S单盘输出ALM 100S上报
//
int save_pge4v_alm(char slot, unsigned char *parg)
{
int num;
unsigned char tmp[14];
memset(tmp, '\0', 14);
num = (int)(slot - 'a');
//
//!屏蔽、告警产生、告警消除
//
rpt_alm_to_client(slot, parg);
sprintf((char *)tmp, "\"%c,j,%s\"", slot, parg);
if((parg[0] >> 4) == 0x05)
memcpy(alm_sta[PGE4S_ALM5_START + num], tmp, strlen(tmp));
else
memcpy(alm_sta[num], tmp, strlen(tmp));
//printf("pge4v2 alarm %s\n",parg);
return 0;
}
int send_init_config(char slot, unsigned char *parg)
{
int num;
printf("###send_init_config\n");
if(slot < 'a' || slot > 'r')
{
return -1;
}
else
{
num = (int)(slot - 'a');
}
printf("###parg:%s\n",parg);
printf("###init_pan:%d\n",num);
init_pan(slot);
return 0;
}
/**********************/
_REVT_NODE REVT_fun[] =
{
{"C23", save_gb_rcv_mode},
{"C25", save_gb_work_mode},
{"C26", save_gb_mask},
{"C01", save_rb_msmode},
{"C02", save_rb_src},
{"CF1", save_gb_rcv_mode},
{"CF2", save_gb_mask},
{"CF3", save_gbtp_sta},
{"CF4", save_gb_tod},
{"CF5", save_gbtp_delay},
{"CF6", save_pps_delay},
{"C24", save_gb_bdzb},
{"C05", save_rb_prio},
{"C06", save_rb_mask},
{"C07", save_rf_tzo},
{"C08", save_rb_dey_chg},
{"C09", save_rb_sta_chg},
{"C03", save_rb_leap_chg},
{"C04", save_rb_leap_flg},
{"C31", save_tod_sta},
{"C41", save_out_lev},
{"C42", save_out_typ},
{"C43", save_out_sta},
{"C44", save_out_prr},
{"C51", save_ptp_net},
{"C52", save_ptp_mod},
{"C14", save_ptp_mtc16}, //ptp mtc ip
{"C53", save_ptp_mtc},
{"CC7", save_ptp_sta}, /*20170616 add */
{"CC9", save_Leap_sta}, /*Satellite leap change*/
{"CC8", save_ptp_dom}, /*20170616 add */
{"C61", save_ref_prio},
{"C62", save_ref_mod},
{"C63", save_ref_sa},
{"C64", save_ref_tl},
{"C65", save_ref_en},
{"C67", save_ref_ssm_en},
{"C71", save_rs_en},
{"C72", save_rs_tzo},
{"C81", save_ppx_mod},
{"CA1", save_tod_en},
{"CA2", save_tod_pro},
{"CB1", save_igb_en},
{"CB2", save_igb_rat},
{"CB3", save_igb_max},
{"CC1", save_ntp_pnet},
{"CC2", save_ntp_en},
{"CC3", save_ntp_key},
{"CC4", save_ntp_ms},
{"CC5", save_ntp_sta},
{"CC6", save_ntp_psta},
{"CD1", save_pgein_net},
{"CD2", save_pgein_mod},
{"CD3", save_pgein_clkclass},
{"CE1", save_pge4v_net},
{"CE2", save_pge4v_mod},
{"CE3", save_pge4v_mtc},
{"CE4", save_pge4v_sta},
{"C84", save_2mb_lvlm}, /*new add*/
{"C10", save_rb_sa},
{"C12", save_ok},
{"C13", save_ok}
};
int NUM_REVT = ((int)(sizeof(REVT_fun) / sizeof(_REVT_NODE)));
_RPT100S_NODE RPT100S_fun[] =
{
{"R-GB-STA", 8, "0", save_gb_sta},
{"R-GB-ORI", 8, "C28", save_gb_ori},
{"R-GB-REV", 8, "C27", save_gb_rev},
{"R-GB-TOD", 8, "C29", save_gb_tod},
{"R-GB-ALM", 8, "0", save_gb_alm}, //GB ALARM
{"R-GBTP-STA",10,"0",save_gbtp_sta}, //GBTPV2 Status.R-GBTP-STA
{"R-GBTP-PST",10,"0",save_gbtp_pst}, //GBTPV2 Postion.R-GBTP-PST
{"R-GBTP-INFO",11,"0", save_gbtp_info}, //GBTPV2 Sat info.R-GBTP-INFO
{"R-GBTP-ALM",10,"0", save_gbtp_alm}, //GBTPV2 ALM.R-GBTP-ALM
{"R-GBTP-DLY",10,"0", save_gbtp_delay}, //GBTPIII DLY.R-GBTP-DLY
{"R-PPS-DLY",9,"0", save_pps_delay}, //GBTPIII DLY.R-PPS-DLY
{"R-GB-MASK", 9, "0", save_gb_mask},
{"R-SY-VER", 8, "0", save_sy_ver},
{"R-RX-DPH", 8, "C00", save_rx_dph},
{"R-RF-SRC", 8, "0", save_rf_src},
{"R-RB-TOD", 8, "0", save_rb_tod},
{"R-RF-DEY", 8, "0", save_rf_dey},
{"R-RF-TZO", 8, "0", save_rf_tzo},
{"R-RX-STA", 8, "0", save_rx_sta},
{"R-RX-ALM", 8, "0", save_rx_alm},
{"R-TOD-STA", 9, "0", save_tod_sta},
{"R-TP-ALM", 8, "0", save_tp_alm},
{"R-OUT-LEV", 9, "0", save_out_lev},
{"R-OUT-TYP", 9, "0", save_out_typ},
{"R-OUT-ALM", 9, "0", save_out_alm},//OUT32 ALARM
{"R-OUT-STA", 9, "0", save_out_sta},
{"R-PTP-NET", 9, "0", save_ptp_net},
{"R-PTP-MOD", 9, "0", save_ptp_mod},
{"R-PTP-ALM", 9, "0", save_ptp_alm},//R-PTP-ALM
{"R-REF-PRIO", 10, "0", save_ref_prio},
{"R-REF-MOD", 9, "0", save_ref_mod},
{"R-REF-PH", 8, "C66", save_ref_ph},
{"R-REF-STA", 9, "0", save_ref_sta},
{"R-REF-ALM", 9, "0", save_ref_alm},//REF ALM
{"R-REF-TPY", 9, "0", save_ref_tpy},
{"R-REF-IEN", 9, "0", save_ref_ssm_en},
{"R-RS-EN", 7, "0", save_rs_en},
{"R-RS-TZO", 8, "0", save_rs_tzo},
{"R-PPX-MOD", 9, "0", save_ppx_mod},
{"R-TOD-EN", 8, "0", save_tod_en},
{"R-TOD-PRO", 9, "0", save_tod_pro},
{"R-IGB-EN", 8, "0", save_igb_en},
{"R-IGB-RAT", 9, "0", save_igb_rat},
{"R-IGB-MAX", 9, "0", save_igb_max},
{"R-IGB-ZONE", 10, "0", save_igb_tzone},/*igb zone set******/
{"R-NTP-STA", 9, "0", save_ntp_sta},
{"R-NTP-NET", 9, "0", save_ntp_net},
{"R-NTP-PNET", 10, "0", save_ntp_pnet},
{"R-NTP-EN", 8, "0", save_ntp_en},
{"R-NTP-PSTA",10, "0", save_ntp_psta},
{"R-NTP-KEY", 9, "0", save_r_ntp_key},
{"R-NTP-MS", 8, "0", save_ntp_ms},
{"R-NTP-ALM", 9, "0", save_ntp_alm},//NTP ALM
{"R-OUT-CFG", 9, "0", save_ext_bid},
{"R-OUT-MGR", 9, "0", save_ext_mgr_pr},
{"R-LEAP-ALM", 10, "0", save_leap_alm},
{"R-STA1-ALM", 10, "0", save_sta1_alm},
{"R-PGEIN-NET", 11, "0", save_pgein_net},
{"R-PGEIN-MOD", 11, "0", save_pgein_mod},
{"R-PGEIN-ALM", 11, "0", save_pgein_alm},
{"R-PGE4V-NET", 11, "0", save_pge4v_net},
{"R-PGE4V-MOD", 11, "0", save_pge4v_mod},
{"R-PGE4V-MTC", 11, "0", save_pge4v_mtc},
{"R-PGE4V-STA", 11, "0", save_pge4v_sta},
{"R-PGE4V-ALM", 11, "0", save_pge4v_alm},
{"R-CONFIG", 8, "0", send_init_config} /*20150909 init pan*/
};
int _NUM_RPT100S = ((int)(sizeof(RPT100S_fun) / sizeof(_RPT100S_NODE))); //2014.12.5
<file_sep>/MCPU/ext_global.h
#ifndef __EXT_GLOBAL__
#define __EXT_GLOBAL__
#include "ext_ctx.h"
extern struct extctx gExtCtx;
#endif//__EXT_GLOBAL__
<file_sep>/MCPU/ext_type.h
#ifndef __EXT_TYPE__
#define __EXT_TYPE__
typedef unsigned char u8_t;
typedef signed char s8_t;
typedef unsigned short u16_t;
typedef signed short s16_t;
typedef unsigned int u32_t;
typedef signed int s32_t;
typedef unsigned long long u64_t;
typedef signed long long s64_t;
typedef enum {false = 0, true = 1} bool_t;
//0~31
#define BIT(i) (1<<(i))
#endif//__EXT_TYPE__
<file_sep>/MCPU/ext_crpt.h
#ifndef __EXT_CRPT__
#define __EXT_CRPT__
#include "ext_ctx.h"
u8_t ext_out_ssm_crpt(u8_t save_flag, u8_t ext_sid, u8_t *ext_data, struct extctx *ctx);
u8_t ext_out_signal_crpt(u8_t save_flag, u8_t ext_sid, u8_t *ext_data, struct extctx *ctx);
u8_t ext_out_mode_crpt(u8_t save_flag, u8_t ext_sid, u8_t *ext_data, struct extctx *ctx);
u8_t ext_out_prr_crpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx);
u8_t ext_out_alm_crpt(u8_t ext_sid, u8_t *ext_data, struct extctx *ctx);
#endif//__EXT_CRPT__
<file_sep>/MCPU/Makefile
#arm-elf-gcc -DMEMWATCH -DMW_STDIO
export CC := arm-elf-gcc
export CFLAGS := -Wall -O3 -pipe
TARGET := mcpu
LOCAL_OBJS := $(patsubst %.c, %.o, $(wildcard *.c))
.PHONY : all
all : $(TARGET)
$(TARGET) : $(LOCAL_OBJS)
$(CC) $(CFLAGS) -o $@ $+ -elf2flt -lpthread
%.o : %.c
$(CC) -c $(CFLAGS) $< -o $@
.PHONY : clean
clean :
rm -f $(TARGET) *.o *.gch *.gdb *.elf *.elf2flt *.bak
<file_sep>/MCPU/mcp_process.c
#include <sys/ipc.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/msg.h>
//#include <sys/select.h>
//#include <sys/time.h>
#include <signal.h>
#include <sys/ioctl.h>
#include <arpa/inet.h>
#include <net/if.h>
#include <net/if_arp.h>
#include <net/route.h>
#include <ctype.h>
#include <unistd.h>
#include <pthread.h>
#include "ext_cmn.h"
#include "ext_global.h"
#include "ext_crpt.h"
#include "ext_trpt.h"
#include "ext_alloc.h"
#include "mcp_process.h"
#include "mcp_def.h"
#include "mcp_set_struct.h"
#include "mcp_save_struct.h"
//#include "memwatch.h"
#define MGE_RALM_NUM 4
int shake_hand_count = 0;
int reset_network_card = 0;
//int _CMD_NODE_MAX = ((int)(sizeof(g_cmd_fun)/sizeof(_CMD_NODE)));
const char *g_cmd_fun_ret[] = { "ENEQ", "IIAC", "ICNV", "IDNV",
"IISP", "IITA", "INUP", "SCSN", "IPMS", "PSWD"
};
unsigned char ssm_alm_last = 0;//0表示无告警
unsigned char ssm_alm_curr = 0;//0表示无告警
void strncpynew(unsigned char *str1, unsigned char *str2, int n)
{
strncpy(str1, str2, n);
*(str1 + n) = '\0';
}
void strncatnew(unsigned char *str1, unsigned char *str2, int n)
{
Uint16 strlength;
strlength = strlen(str1);
strncat(str1, str2, n);
*(str1 + strlength + n) = '\0';
}
int strncmp1(unsigned char *p1, unsigned char *p2, unsigned char n)
{
unsigned int i = 0;
signed char j = 0;
for (i = 0; i < n; i++)
{
if (p1[i] == p2[i])
{
;
}
else if(p1[i] > p2[2])
{
j = 1;
break;
}
else
{
j = -1;
break;
}
}
return j;
}
void timefunc(int sig)
{
int count, i;
int client_num[MAX_CLIENT];
count = judge_client_online(client_num);
for(i = 0; i < count; i++)
{
if(timeout_cnt[client_num[i]] == 0)
{
FD_CLR(client_fd[client_num[i]], &allset);
close(client_fd[client_num[i]]);
client_fd[client_num[i]] = -1;
printf("<timeout>close client<%d>\n", client_num[i]);
}
timeout_cnt[client_num[i]] = 0;
}
reset_network_card = 1;
}
int init_timer()
{
struct itimerval value;
memset(&value, 0, sizeof(struct itimerval));
value.it_value.tv_sec = 120;
value.it_interval.tv_sec = 120;
signal(SIGALRM, timefunc); /*捕获定时信号 */
setitimer(ITIMER_REAL, &value, NULL); /*定时开始 */
//printf("250s timer startx\n");
return 0;
}
int msgQ_create( const char *pathname, char proj_id )
{
key_t key;
int msgQ_id;
/* Create unique key via call to ftok() */
key = ftok( pathname, proj_id );
if ( -1 == key )
{
return(-1);
}
/* Open the queue,create if necessary */
msgQ_id = msgget( key, IPC_CREAT | 0660 );
if( -1 == msgQ_id )
{
return (-1);
}
#if DEBUG_NET_INFO
printf("%s:qid=%d\n", NET_INFO, msgQ_id);
#endif
return (msgQ_id);
}
int almMsk_id(int index, char type)
{
if(type != conf_content.alm_msk[index].type)
{
conf_content.alm_msk[index].type = type;
return 0;
}
else
{
return 1;
}
}
void init_almMsk(void)
{
if(almMsk_id(0, 'L') == 0 )//GBTP Y0 Y1,
{
memset(conf_content.alm_msk[0].data, 0x4f, 2);
memset(conf_content.alm_msk[0].data + 2, 0, 22);
conf_content.alm_msk[0].data[0] = 0x4b;
}
else
{
memset(conf_content.alm_msk[0].data + 2, 0, 22);
conf_content.alm_msk[0].data[0] &= ~(1 << 2); //alrm mask bit 2 ,1pps+tod alarm.
}
if(almMsk_id(1, 'g') == 0 )//GBTP2
{
memset(conf_content.alm_msk[1].data, 0x4f, 2);
memset(conf_content.alm_msk[1].data + 2, 0, 22);
conf_content.alm_msk[1].data[0] = 0x4b;
}
else
{
memset(conf_content.alm_msk[1].data + 2, 0, 22);
conf_content.alm_msk[1].data[0] &= ~(1 << 2); // alrm mask bit 2 ,1pps+tod alarm.
}
if(almMsk_id(2, 'P') == 0 )//GTP
{
memset(conf_content.alm_msk[2].data, 0x4f, 2);
memset(conf_content.alm_msk[2].data + 2, 0, 22);
conf_content.alm_msk[2].data[0] = 0x49;
}
else
{
memset(conf_content.alm_msk[2].data + 2, 0, 22);
conf_content.alm_msk[2].data[0] &= ~(3 << 1);
}
if(almMsk_id(3, 'e') == 0 )//GGTP
{
memset(conf_content.alm_msk[3].data, 0x4f, 2);
memset(conf_content.alm_msk[3].data + 2, 0, 22);
conf_content.alm_msk[3].data[0] = 0x4b;
}
else
{
memset(conf_content.alm_msk[3].data + 2, 0, 22);
conf_content.alm_msk[3].data[0] &= ~(1 << 2);
}
if(almMsk_id(4, 'l') == 0 )//BTP
{
memset(conf_content.alm_msk[4].data, 0x4f, 2);
memset(conf_content.alm_msk[4].data + 2, 0, 22);
conf_content.alm_msk[4].data[0] = 0x4a;
}
else
{
memset(conf_content.alm_msk[4].data + 2, 0, 22);
conf_content.alm_msk[4].data[0] &= ~(1 << 0);
conf_content.alm_msk[4].data[0] &= ~(1 << 2);
}
if(almMsk_id(5, 'K') == 0 )//RB
{
memset(conf_content.alm_msk[5].data, 0x4f, 5);
memset(conf_content.alm_msk[5].data + 5, 0, 19);
conf_content.alm_msk[5].data[0] = 0x43; // Mask 17# 18# 1pp+tod
conf_content.alm_msk[5].data[2] = 0x4b; //
conf_content.alm_msk[5].data[4] = 0x4e;
conf_content.alm_msk[5].data[1] &= ~(0x3 << 2);// mask LOF、AIS
conf_content.alm_msk[5].data[2] &= ~(0x7 << 0);// mask BPV、CRC
}
else
{
memset(conf_content.alm_msk[5].data + 5, 0, 19);
conf_content.alm_msk[5].data[0] &= ~( (1 << 2) | (1 << 3) );//mask 17# 18# 1pps+tod
//conf_content.alm_msk[4].data[2] &= ~(1 << 2);//modify:2017-07-19, delete the Null mask.
conf_content.alm_msk[5].data[4] &= ~1;
conf_content.alm_msk[5].data[1] &= ~(0x3 << 2);
conf_content.alm_msk[5].data[2] &= ~(0x7 << 0);
}
if(almMsk_id(6, 'R') == 0 )
{
memset(conf_content.alm_msk[6].data, 0x4f, 5);
memset(conf_content.alm_msk[6].data + 5, 0, 19);
conf_content.alm_msk[6].data[0] = 0x43;
conf_content.alm_msk[6].data[2] = 0x4b;
conf_content.alm_msk[6].data[4] = 0x4e;
}
else
{
memset(conf_content.alm_msk[6].data + 5, 0, 19);
conf_content.alm_msk[6].data[0] &= ~( (1 << 2) | (1 << 3) );
//conf_content.alm_msk[5].data[2] &= ~(1 << 2);//modify:2017-07-19, delete the Null mask.
conf_content.alm_msk[6].data[4] &= ~1;
}
if(almMsk_id(7, 'I') == 0 )//TP16
{
memset(conf_content.alm_msk[7].data, 0x4f, 6);
memset(conf_content.alm_msk[7].data + 6, 0, 18);
conf_content.alm_msk[7].data[0] = 0x40;
}
else
{
memset(conf_content.alm_msk[7].data + 6, 0, 18);
//conf_content.alm_msk[7].data[0] = 0x40;
}
if(almMsk_id(8, 'J') == 0 )//OUT16
{
memset(conf_content.alm_msk[8].data, 0x4f, 6);
memset(conf_content.alm_msk[8].data + 6, 0, 18);
}
else
{
memset(conf_content.alm_msk[8].data + 6, 0, 18);
}
if(almMsk_id(9, 'a') == 0 )//OUT32
{
memset(conf_content.alm_msk[9].data, 0x4f, 6);
memset(conf_content.alm_msk[9].data + 6, 0, 18);
}
else
{
memset(conf_content.alm_msk[9].data + 6, 0, 18);
}
if(almMsk_id(10, 'b') == 0 )//OUTH32
{
memset(conf_content.alm_msk[10].data, 0x4f, 6);
memset(conf_content.alm_msk[10].data + 6, 0, 18);
}
else
{
memset(conf_content.alm_msk[10].data + 6, 0, 18);
}
if(almMsk_id(11, 'c') == 0 )//OUTE32
{
memset(conf_content.alm_msk[11].data, 0x4f, 6);
memset(conf_content.alm_msk[11].data + 6, 0, 18);
}
else
{
memset(conf_content.alm_msk[11].data + 6, 0, 18);
}
/*if(almMsk_id(11, 'A') == 0 )//PTP4
{
memset(conf_content.alm_msk[11].data, 0x4f, 3);
memset(conf_content.alm_msk[11].data + 3, 0, 21);
conf_content.alm_msk[11].data[0] = 0x40;
}
else
{
memset(conf_content.alm_msk[11].data + 3, 0, 21);
conf_content.alm_msk[11].data[0] = 0x40;
}
if(almMsk_id(12, 'B') == 0 )//PF04
{
memset(conf_content.alm_msk[12].data, 0x4f, 3);
memset(conf_content.alm_msk[12].data + 3, 0, 21);
conf_content.alm_msk[12].data[0] = 0x40;
}
else
{
memset(conf_content.alm_msk[12].data + 3, 0, 21);
conf_content.alm_msk[12].data[0] = 0x40;
}
if(almMsk_id(13, 'C') == 0 )//PGE4
{
memset(conf_content.alm_msk[13].data, 0x4f, 3);
memset(conf_content.alm_msk[13].data + 3, 0, 21);
conf_content.alm_msk[13].data[0] = 0x40;
}
else
{
memset(conf_content.alm_msk[13].data + 3, 0, 21);
conf_content.alm_msk[13].data[0] = 0x40;
}
if(almMsk_id(14, 'D') == 0 )//PGO4
{
memset(conf_content.alm_msk[14].data, 0x4f, 3);
memset(conf_content.alm_msk[14].data + 3, 0, 21);
conf_content.alm_msk[14].data[0] = 0x40;
}
else
{
memset(conf_content.alm_msk[14].data + 3, 0, 21);
conf_content.alm_msk[14].data[0] = 0x40;
}*/
if(almMsk_id(12, 'S') == 0 )//REFTF
{
memset(conf_content.alm_msk[12].data, 0x4f, 6);
memset(conf_content.alm_msk[12].data + 6, 0x5f, 6);
memset(conf_content.alm_msk[12].data + 12, 0, 12);
}
else
{
memset(conf_content.alm_msk[12].data + 12, 0, 12);
}
if(almMsk_id(13, 'U') == 0 )//PPX
{
memset(conf_content.alm_msk[13].data, 0x40, 1);
memset(conf_content.alm_msk[13].data + 1, 0, 23);
}
else
{
memset(conf_content.alm_msk[13].data + 1, 0, 23);
//conf_content.alm_msk[13].data[0] = 0x40;
}
if(almMsk_id(14, 'V') == 0 )//TOD16
{
memset(conf_content.alm_msk[14].data, 0x40, 1);
memset(conf_content.alm_msk[14].data + 1, 0, 23);
}
else
{
memset(conf_content.alm_msk[14].data + 1, 0, 23);
//conf_content.alm_msk[14].data[0] = 0x40;
}
if(almMsk_id(15, 'Z') == 0 )//NTP12
{
memset(conf_content.alm_msk[15].data, 0x4f, 4);
memset(conf_content.alm_msk[15].data + 4, 0, 20);//modify:2017-07-19 ,alarm mask,
}
else
{
memset(conf_content.alm_msk[15].data + 4, 0, 20);//modify:2017-07-19,alarm mask.
}
if(almMsk_id(16, 'N') == 0 )//MCP
{
memset(conf_content.alm_msk[16].data, 0x4f, 6);
//memset(conf_content.alm_msk[16].data + 6, 0x5f, 6);
memset(conf_content.alm_msk[16].data + 6, 0, 18);
conf_content.alm_msk[16].data[0] &= ~( (1 << 2) | (1 << 3) );
conf_content.alm_msk[16].data[1] &= ~( 1 << 3) ;
//conf_content.alm_msk[19].data[2] = 0x48;
//memset(conf_content.alm_msk[19].data + 3, 0x40, 3);
}
else
{
memset(conf_content.alm_msk[16].data + 6, 0, 18);
conf_content.alm_msk[16].data[0] &= ~( (1 << 2) | (1 << 3) );
conf_content.alm_msk[16].data[1] &= ~(1 << 3);
//memset(conf_content.alm_msk[19].data + 2, 0x40, 4);
}
if(almMsk_id(17, 'Q') == 0 )//FAN
{
memset(conf_content.alm_msk[17].data, 0x4f, 1);
memset(conf_content.alm_msk[17].data + 1, 0, 23);
}
else
{
memset(conf_content.alm_msk[17].data + 1, 0, 23);
}
if(almMsk_id(18, 'h') == 0 )//out32s
{
memset(conf_content.alm_msk[18].data, 0x4f, 6);
memset(conf_content.alm_msk[18].data + 6, 0, 18);
}
else
{
memset(conf_content.alm_msk[18].data + 6, 0, 18);
}
if(almMsk_id(19, 'i') == 0 )//OUT16S
{
memset(conf_content.alm_msk[19].data, 0x4f, 6);
memset(conf_content.alm_msk[19].data + 6, 0, 18);
}
else
{
memset(conf_content.alm_msk[19].data + 6, 0, 18);
}
if(almMsk_id(20, 's') == 0 )//BPGEIN
{
memset(conf_content.alm_msk[20].data, 0x4f, 6);
memset(conf_content.alm_msk[20].data + 6, 0, 18);
}
else
{
memset(conf_content.alm_msk[20].data + 6, 0, 18);
}
if(almMsk_id(21, 'j') == 0 )//pge4v2
{
memset(conf_content.alm_msk[21].data, 0x4f, 6);
memset(conf_content.alm_msk[21].data + 6, 0x5f, 6);
memset(conf_content.alm_msk[21].data + 12, 0, 12);
}
else
{
memset(conf_content.alm_msk[21].data + 12, 0, 12);
}
if(almMsk_id(22, 'v') == 0 )//GBTPIIV2
{
memset(conf_content.alm_msk[22].data, 0x4f, 2);
memset(conf_content.alm_msk[22].data + 2, 0, 22);
}
else
{
memset(conf_content.alm_msk[22].data + 2, 0, 22);
}
if(almMsk_id(23, 'f') == 0 )//MGE
{
memset(conf_content.alm_msk[23].data, 0x4f, 4);
memset(conf_content.alm_msk[23].data + 4, 0, 20);
}
else
{
memset(conf_content.alm_msk[23].data + 4, 0, 20);
}
if(almMsk_id(24, 'd') == 0 )//DRV
{
memset(conf_content.alm_msk[24].data, 0x4f, 1);
memset(conf_content.alm_msk[24].data + 1, 0, 23);
}
else
{
memset(conf_content.alm_msk[24].data + 1, 0, 23);
}
memset(&(conf_content.alm_msk[25]), 0, (alm_msk_total - 26)*sizeof(struct ALM_MSK));
}
void init_var(void)
{
int i;
memset(slot_type, 'O', sizeof(unsigned char) * 24);
slot_type[20] = 'N';
//
//!clear temp alarm cache.
//
memset(&flag_alm, '\0', sizeof(int) * 36 * 14);
memset(online_sta, 0, sizeof(int) * 24);
//
//!clear alarm array.
//
memset(&alm_sta, '\0', sizeof(unsigned char) * 37 * 14);
memset(fpga_ver, '\0', 7);
memset(timeout_cnt, 0, sizeof(int) * 4);
//printf("%s\n",slot_type);
memset(&saved_alms, 0, SAVE_ALM_NUM * 50);
saved_alms_cnt = 0;
memcpy(mcp_date, "2011-11-11", 10);
memcpy(mcp_time, "11:11:11", 8);
memcpy(password, "<PASSWORD>", 8);
memcpy(alm_sta[20], "\"u,N,@@@\"", 9);
g_DrvLost = 200;
i = 0;
i = atoi(conf_content.slot_u.leap_num);
if(strncmp1(conf_content.slot_u.leap_num, "00", 2) == 0)
{}
else if((i < 1) || (i > 99))
{
memcpy(conf_content.slot_u.leap_num, "18", 2);
}
/****test**/
for(i = 0; i < 14; i++)
{
rpt_content.slot[i].out_mode = '0';
rpt_content.slot[i].out_sta = '0';
}
/*
printf("slot_type=<%s>\n",slot_type);
printf("online=<");
for(i=0;i<24;i++)
printf("%d",online_sta[i]);
printf(">;\n");
*/
atag = 0;
flag_i_o = 0;
flag_i_p = 0;
time_temp = 0;
current_client = -1;
g_lev = -1;
qid = -1;
rpt_content.slot_o.rb_sa = '@';
rpt_content.slot_p.rb_sa = '@';
rpt_content.slot_o.rb_tl = 'g';
rpt_content.slot_p.rb_tl = 'g';
rpt_content.slot_o.rb_tl_2mhz = 'g';
rpt_content.slot_p.rb_tl_2mhz = 'g';
rpt_content.slot[0].ref_2mb_lvlm = '0';
rpt_content.slot[1].ref_2mb_lvlm = '0';
//GBTPIIV2 VAR INIT
rpt_content.slot_q.gb_sta = '0';
rpt_content.slot_q.staen = '1';
rpt_content.slot_q.gb_tod[0] = '3';
rpt_content.slot_q.leapalm[0] = '0';
rpt_content.slot_q.leapalm[1] = '0';
rpt_content.slot_q.leapnum[0] = '0';
rpt_content.slot_q.leapnum[1] = '0';
rpt_content.slot_r.gb_sta = '0';
rpt_content.slot_r.staen = '1';
rpt_content.slot_r.gb_tod[0] = '3';
rpt_content.slot_r.leapalm[0] = '0';
rpt_content.slot_r.leapalm[1] = '0';
rpt_content.slot_r.leapnum[0] = '0';
rpt_content.slot_r.leapnum[1] = '0';
//告警屏蔽初始化;
init_almMsk();
memset(event_old, 0, 17 * 128);
g_LeapFlag = 0;
}
int Create_Thread(bool_t * loop)
{
pthread_t thread1;
pthread_attr_t attr;
pthread_attr_init(&attr);
pthread_attr_setdetachstate(&attr, PTHREAD_CREATE_DETACHED);
if(pthread_create(&thread1,&attr,(void *)timer_mcp,(void *) loop) != 0 ) {
printf("pthread_create1 mcp gf_Usual error.\n");
return(false);
}
pthread_attr_destroy(&attr);
return (true);
}
void init_sta1_ip(unsigned char slot)
{
unsigned char ip_temp[9];
unsigned char gate_temp[9];
unsigned char mask_temp[9];
if( (( (conf_content3.sta1.ip[0] ) & 0xf0 ) != 0x40) ||
(((conf_content3.sta1.ip[2] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.ip[4] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.ip[6] ) & 0xf0) != 0x40) )
{
memset(conf_content3.sta1.ip, 0, 9);
memcpy(conf_content3.sta1.ip, "L@JH@AFC", 8);
}
if( (((conf_content3.sta1.gate[0] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.gate[2] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.gate[4] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.gate[6] ) & 0xf0) != 0x40) )
{
memset(conf_content3.sta1.gate, 0, 9);
memcpy(conf_content3.sta1.gate, "L@JH@A@A", 8);
}
if( (((conf_content3.sta1.mask[0] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.mask[2] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.mask[4] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.mask[6] ) & 0xf0) != 0x40) )
{
memset(conf_content3.sta1.mask, 0, 9);
memcpy(conf_content3.sta1.mask, "OOOOOO@@", 8);
}
memset(ip_temp, 0, 9);
memcpy(ip_temp, conf_content3.sta1.ip, 8);
set_sta_ip(ip_temp, slot);
sleep(1);
memset(gate_temp, 0, 9);
memcpy(gate_temp, conf_content3.sta1.gate, 8);
set_sta_gateway(gate_temp, slot);
sleep(1);
memset(mask_temp, 0, 9);
memcpy(mask_temp, conf_content3.sta1.mask, 8);
set_sta_netmask(mask_temp, slot);
}
void init_sta1(int num, unsigned char *sendbuf)
{
unsigned char slot;
//DevType
slot = 'a' + num;
init_sta1_ip(slot);
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/LLN0/DevType=GNSS-97-P300;");
sendtodown(sendbuf, (char)('a' + num));
sendtodown(sendbuf, (char)('a' + num));
usleep(500);
//DevDescr
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/LLN0/DevDescr=P300;");
sendtodown(sendbuf, (char)('a' + num));
sendtodown(sendbuf, (char)('a' + num));
#if 1
//Company
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/LLN0/Company=DTT;");
sendtodown(sendbuf, (char)('a' + num));
sendtodown(sendbuf, (char)('a' + num));
//PortNum 实际为板卡数量
sta1_portnum(sendbuf, slot);
//HWVersion
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/LLN0/HWVersion=v01.00;");
sendtodown(sendbuf, (char)('a' + num));
sendtodown(sendbuf, (char)('a' + num));
//FWVersion
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/LLN0/FWVersion=v01.00;");
sendtodown(sendbuf, (char)('a' + num));
sendtodown(sendbuf, (char)('a' + num));
//SWVersion
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-STA1:000000::MONT/LLN0/SWVersion=v01.00;");
sendtodown(sendbuf, (char)('a' + num));
sendtodown(sendbuf, (char)('a' + num));
//ip mac
sta1_net(sendbuf, slot);
//CpuUsage
sta1_cpu(sendbuf, slot);
//InType OutType
sta1_in_out(sendbuf, slot);
//Alm
sta1_alm(sendbuf, slot);
#endif
}
void delay_s(int iSec,int iUsec)
{
struct timeval tv;
tv.tv_sec=iSec;
tv.tv_usec=iUsec;
select(0,NULL,NULL,NULL,&tv);
}
void init_pan(char slot)
{
/* RB1*/
unsigned char out_ssm_threshold = 0, out_port = 4;
int j, len, num, flag, len1, len2;
unsigned char sendbuf[SENDBUFSIZE];
unsigned char *ctag = "000000";
unsigned char tmp1[3], tmp2[3], tmp3[3], tmp4[3], tmp5[3], tmp6[3], tmp7[3];
//int flag_lev=0;
num = (int)(slot - 'a');
//printf("###init_pan:%d\n",num);
if(slot == 20) /*mcp*/
{
memset(sendbuf, '\0', SENDBUFSIZE);
num = atoi(conf_content.slot_u.leap_num);
if(strncmp1(conf_content.slot_u.leap_num, "00", 2) == 0)
{
}
else if((num < 1) || (num > 99))
{
return ;
}
sprintf((char *)sendbuf, "SET-LEAP-NUM:%s::%s;", ctag, conf_content.slot_u.leap_num);
sendtodown(sendbuf, 'u');
}
else if(slot == RB1_SLOT)
{
if(slot_type[num] == 'Y')
{
return;
}
if(conf_content.slot_o.msmode[0] != 0xff)
{
if(strlen(conf_content.slot_o.msmode) == 4)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-MAIN:%s::%s;", ctag, conf_content.slot_o.msmode);
sendtodown(sendbuf, 'o');
}
}
if(conf_content.slot_o.sys_ref != 0xff)
{
if(((conf_content.slot_o.sys_ref > 0x29) && (conf_content.slot_o.sys_ref < 0x39))||conf_content.slot_o.sys_ref == 0x46)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-SYS-REF:%s::INP-%c;", ctag, conf_content.slot_o.sys_ref);
sendtodown(sendbuf, 'o');
}
}
if(conf_content.slot_o.priority[0] != 0xff)
{
if(strlen(conf_content.slot_o.priority) == 8)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PRIO-INP:%s::%s;", ctag, conf_content.slot_o.priority);
sendtodown(sendbuf, 'o');
}
}
if(conf_content.slot_o.mask[0] != 0xff)
{
if(strlen(conf_content.slot_o.mask) == 8)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-MASK-INP:%s::%s;", ctag, conf_content.slot_o.mask);
sendtodown(sendbuf, 'o');
}
}
if(conf_content.slot_o.dely[0][0] != 0xff)
{
if(strlen(conf_content.slot_o.dely[0]) < 9)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-DELY:%s::1,%s;", ctag, conf_content.slot_o.dely[0]);
sendtodown(sendbuf, 'o');
}
}
if(conf_content.slot_o.dely[1][0] != 0xff)
{
if(strlen(conf_content.slot_o.dely[1]) < 9)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-DELY:%s::2,%s;", ctag, conf_content.slot_o.dely[1]);
sendtodown(sendbuf, 'o');
}
}
if(conf_content.slot_o.dely[2][0] != 0xff)
{
if(strlen(conf_content.slot_o.dely[2]) < 9)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-DELY:%s::3,%s;", ctag, conf_content.slot_o.dely[2]);
sendtodown(sendbuf, 'o');
}
}
if(conf_content.slot_o.dely[3][0] != 0xff)
{
if(strlen(conf_content.slot_o.dely[3]) < 9)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-DELY:%s::4,%s;", ctag, conf_content.slot_o.dely[3]);
sendtodown(sendbuf, 'o');
}
}
if(conf_content.slot_o.tzo[0] != 0xff)
{
if(strlen(conf_content.slot_o.tzo) == 3)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-TZO:%s::%s;", ctag, conf_content.slot_o.tzo);
sendtodown(sendbuf, 'o');
printf("15 set tzo %s\n",conf_content.slot_o.tzo);
}
}
if(conf_content.slot_o.leaptag != 0xff)
{
if((conf_content.slot_o.leaptag > 0x29) && (conf_content.slot_o.leaptag < 0x34))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-LEAP:%s::%c;", ctag, conf_content.slot_o.leaptag);
sendtodown(sendbuf, 'o');
}
}
if(conf_content.slot_o.leapmask[0] != 0xff)
{
if(strlen(conf_content.slot_o.leapmask) == 5)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-LMAK-INP:%s::%s;", ctag, conf_content.slot_o.leapmask);
sendtodown(sendbuf, 'o');
}
}
}
/*RB2*/
else if(slot == RB2_SLOT)
{
if(slot_type[num] == 'Y')
{
return;
}
if(conf_content.slot_p.msmode[0] != 0xff)
{
if(strlen(conf_content.slot_p.msmode) == 4)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-MAIN:%s::%s;", ctag, conf_content.slot_p.msmode);
sendtodown(sendbuf, 'p');
}
}
if(conf_content.slot_p.sys_ref != 0xff)
{
if(((conf_content.slot_p.sys_ref > 0x29) && (conf_content.slot_p.sys_ref < 0x39))||conf_content.slot_p.sys_ref == 0x46)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-SYS-REF:%s::INP-%c;", ctag, conf_content.slot_p.sys_ref);
sendtodown(sendbuf, 'p');
}
}
if(conf_content.slot_p.priority[0] != 0xff)
{
if(strlen(conf_content.slot_p.priority) == 8)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PRIO-INP:%s::%s;", ctag, conf_content.slot_p.priority);
sendtodown(sendbuf, 'p');
}
}
if(conf_content.slot_p.mask[0] != 0xff)
{
if(strlen(conf_content.slot_p.mask) == 8)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-MASK-INP:%s::%s;", ctag, conf_content.slot_p.mask);
sendtodown(sendbuf, 'p');
}
}
if(conf_content.slot_p.dely[0][0] != 0xff)
{
if(strlen(conf_content.slot_p.dely[0]) < 9)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-DELY:%s::1,%s;", ctag, conf_content.slot_p.dely[0]);
sendtodown(sendbuf, 'p');
}
}
if(conf_content.slot_p.dely[1][0] != 0xff)
{
if(strlen(conf_content.slot_p.dely[1]) < 9)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-DELY:%s::2,%s;", ctag, conf_content.slot_p.dely[1]);
sendtodown(sendbuf, 'p');
}
}
if(conf_content.slot_p.dely[2][0] != 0xff)
{
if(strlen(conf_content.slot_p.dely[2]) < 9)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-DELY:%s::3,%s;", ctag, conf_content.slot_p.dely[2]);
sendtodown(sendbuf, 'p');
}
}
if(conf_content.slot_p.dely[3][0] != 0xff)
{
if(strlen(conf_content.slot_p.dely[3]) < 9)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-DELY:%s::4,%s;", ctag, conf_content.slot_p.dely[3]);
sendtodown(sendbuf, 'p');
}
}
if(conf_content.slot_p.tzo[0] != 0xff)
{
if(strlen(conf_content.slot_p.tzo) == 3)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-TZO:%s::%s;", ctag, conf_content.slot_p.tzo);
sendtodown(sendbuf, 'p');
printf("16 set tzo %s\n",conf_content.slot_p.tzo);
}
}
if(conf_content.slot_p.leaptag != 0xff)
{
if((conf_content.slot_p.leaptag > 0x29) && (conf_content.slot_p.leaptag < 0x34))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-LEAP:%s::%c;", ctag, conf_content.slot_p.leaptag);
sendtodown(sendbuf, 'p');
}
}
if(conf_content.slot_p.leapmask[0] != 0xff)
{
if(strlen(conf_content.slot_p.leapmask) == 5)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-LMAK-INP:%s::%s;", ctag, conf_content.slot_p.leapmask);
sendtodown(sendbuf, 'p');
}
}
}
/*gbtp1*/
else if(slot == GBTP1_SLOT)
{
if(slot_type[num] == 'Y')
{
return;
}
if(conf_content.slot_q.mode[0] != 0xff)
{
if( ('L' == slot_type[16]) ||
('e' == slot_type[16]) ||
'l' == slot_type[16] ||
'g' == slot_type[16]
)
{
len = strlen(conf_content.slot_q.mode);
if( (2 == len) || (3 == len) )
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-MODE:%s::%s;", ctag, conf_content.slot_q.mode);
sendtodown(sendbuf, 'q');
}
}
else if( 'P' == slot_type[16] )
{
if( 0 == strncmp1(conf_content.slot_q.mode, "GPS", 3) )
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-MODE:%s::%s;", ctag, conf_content.slot_q.mode);
sendtodown(sendbuf, 'q');
}
}
else if('v' == slot_type[16] || 'x' == slot_type[16] )
{
len = strlen(conf_content.slot_q.mode);
if((2 == len)||(3 == len))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-GBTP-MODE:%s::%s;", ctag, conf_content.slot_q.mode);
sendtodown(sendbuf, 'q');
}
}
}/*
if(conf_content.slot_q.pos[0]!=0xff)
{
if(strlen(conf_content.slot_q.pos)>35)
{
memset(sendbuf,'\0',SENDBUFSIZE);
sprintf((char *)sendbuf,"SET-POS:%s::%s;",ctag,conf_content.slot_q.pos);
sendtodown(sendbuf,'q');
}
}*/
if('v' == slot_type[16])//SET-GBTP-SATEN
{
if((conf_content.slot_q.mask[0] == '0') ||(conf_content.slot_q.mask[0] == '1'))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-GBTP-SATEN:%s::%s;", ctag, conf_content.slot_q.mask);
sendtodown(sendbuf, 'q');
}
}
else if('x' == slot_type[16])
{
if((conf_content.slot_q.mask[0] == '0') ||(conf_content.slot_q.mask[0] == '1'))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-GBTP-SATEN:%s::%s;", ctag, conf_content.slot_q.mask);
sendtodown(sendbuf, 'q');
}
if((strlen(conf_content3.gbtp_delay[0]) <=6) &&
(atoi(conf_content3.gbtp_delay[0]) <= 32767) &&
(atoi(conf_content3.gbtp_delay[0]) >= -32768))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-GBTP-DELAY:%s::%s;", ctag, conf_content3.gbtp_delay[0]);
sendtodown(sendbuf, 'q');
}
if((strlen(conf_content3.gbtp_pps_delay[0]) <=10) &&
(atoi(conf_content3.gbtp_pps_delay[0]) <= 200000000) &&
(atoi(conf_content3.gbtp_pps_delay[0]) >= -200000000))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PPS-DELAY:%s::%s;", ctag, conf_content3.gbtp_pps_delay[0]);
sendtodown(sendbuf, 'q');
}
}
else
{
if(conf_content.slot_q.mask[0] != 0xff)
{
if(strlen(conf_content.slot_q.mask) == 2)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-MASK:%s::%s;", ctag, conf_content.slot_q.mask);
sendtodown(sendbuf, 'q');
}
}
}
}
/*gbtp2*/
else if(slot == GBTP2_SLOT)
{
if(slot_type[num] == 'Y')
{
return;
}
if(conf_content.slot_r.mode[0] != 0xff)
{
if( ('L' == slot_type[17]) ||
('e' == slot_type[17]) ||
'l' == slot_type[17] ||
'g' == slot_type[17]
)
{
len = strlen(conf_content.slot_r.mode);
if( (2 == len) || (3 == len) )
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-MODE:%s::%s;", ctag, conf_content.slot_r.mode);
sendtodown(sendbuf, 'r');
}
}
else if( 'P' == slot_type[17])
{
if( 0 == strncmp1(conf_content.slot_r.mode, "GPS", 3) )
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-MODE:%s::%s;", ctag, conf_content.slot_r.mode);
sendtodown(sendbuf, 'r');
}
}
else if('v' == slot_type[17] || 'x' == slot_type[17])
{
len = strlen(conf_content.slot_r.mode);
printf("enter init pan 18\r\n");
if((2 == len)||(3 == len))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-GBTP-MODE:%s::%s;", ctag, conf_content.slot_r.mode);
sendtodown(sendbuf, 'r');
printf(sendbuf);
}
}
}/*
if(conf_content.slot_r.pos[0]!=0xff)
{
len=strlen(conf_content.slot_r.pos);
if((len<35)&&(len>25))
{
memset(sendbuf,'\0',SENDBUFSIZE);
sprintf((char *)sendbuf,"SET-POS:%s::%s;",ctag,conf_content.slot_r.pos);
sendtodown(sendbuf,'r');
}
}*/
if('v' == slot_type[17] )//SET-GBTP-SATEN
{
if((conf_content.slot_r.mask[0] == '0') ||(conf_content.slot_r.mask[0] == '1'))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-GBTP-SATEN:%s::%s;", ctag, conf_content.slot_r.mask);
sendtodown(sendbuf, 'r');
}
}
else if('x' == slot_type[17])
{
if((conf_content.slot_r.mask[0] == '0') ||(conf_content.slot_r.mask[0] == '1'))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-GBTP-SATEN:%s::%s;", ctag, conf_content.slot_r.mask);
sendtodown(sendbuf, 'r');
}
if((strlen(conf_content3.gbtp_delay[1]) <=6) &&
(atoi(conf_content3.gbtp_delay[1]) <= 32767) &&
(atoi(conf_content3.gbtp_delay[1]) >= -32768))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-GBTP-DELAY:%s::%s;", ctag, conf_content3.gbtp_delay[1]);
sendtodown(sendbuf, 'r');
}
if((strlen(conf_content3.gbtp_pps_delay[1]) <=10) &&
(atoi(conf_content3.gbtp_pps_delay[1]) <= 200000000) &&
(atoi(conf_content3.gbtp_pps_delay[1]) >= -200000000))
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PPS-DELAY:%s::%s;", ctag, conf_content3.gbtp_pps_delay[1]);
sendtodown(sendbuf, 'r');
}
}
else
{
if(conf_content.slot_r.mask[0] != 0xff)
{
if(strlen(conf_content.slot_r.mask) == 2)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-MASK:%s::%s;", ctag, conf_content.slot_r.mask);
sendtodown(sendbuf, 'r');
}
}
}
}
/*out*/
else if((num >= 0) && (num < 14))
{
if( (slot_type[num] == 'J') ||
(slot_type[num] == 'a') ||
(slot_type[num] == 'b') ||
(slot_type[num] == 'c') ||
(slot_type[num] == 'h') ||
(slot_type[num] == 'i'))
{
/*if(conf_content.slot[num].out_lev[0]!=0xff)
{
if(strlen(conf_content.slot[num].out_lev)==2)
{
memset(sendbuf,'\0',SENDBUFSIZE);
sprintf((char *)sendbuf,"SET-OUT-LEV:%s::%s;",ctag,conf_content.slot[num].out_lev);
sendtodown(sendbuf,(char)('a'+num));
}
}*/
//
//OUT32S ADD
//
//printf("###init_pan:%d\n",num);
get_out_lev(num, 1);
//printf("test\n");
if(conf_content.slot[num].out_typ[0] != 0xff)
{
if(strlen(conf_content.slot[num].out_typ) == 16)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-OUT-TYP:%s::%s;", ctag, conf_content.slot[num].out_typ);
sendtodown(sendbuf, (char)('a' + num));
//
//OUT32S ADD.
//
//printf("out32s:%s\n",sendbuf);
}
}
if(conf_content.slot[num].out_mod != 0xff)
{
if( (conf_content.slot[num].out_mod != '0') && (conf_content.slot[num].out_mod != '1'))
conf_content.slot[num].out_mod = '0';
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-OUT-MOD:%s::%c;", ctag, conf_content.slot[num].out_mod);
sendtodown(sendbuf, (char)('a' + num));
//
//OUT32S ADD
//
//printf("out32s:%s\n",sendbuf);
}
}
//
//! tp16 initialtion.
//
else if(slot_type[num] == 'I')
{
if(conf_content.slot[num].tp16_en[0] != 0xff)
{
if(strlen(conf_content.slot[num].tp16_en) == 16)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-TP16-EN:%s::%s;", ctag, conf_content.slot[num].tp16_en);
sendtodown(sendbuf, (char)('a' + num));
}
}
j = atoi(conf_content.slot_u.leap_num);
if(strncmp1(conf_content.slot_u.leap_num, "00", 2) == 0)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-TP16-LEAP:%s::%s;", ctag, conf_content.slot_u.leap_num);
sendtodown(sendbuf, (char)('a' + num));
}
else if((j < 1) || (j > 99))
{}
else
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-TP16-LEAP:%s::%s;", ctag, conf_content.slot_u.leap_num);
sendtodown(sendbuf, (char)('a' + num));
printf("set tp16 leap %d\n",j);
}
//2015.9.9 初始化TP16协议
if( '0' == conf_content3.mcp_protocol ||
'1' == conf_content3.mcp_protocol ||
'2' == conf_content3.mcp_protocol )
{
printf("###init protocol ptp --%d\n",num);
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PROTOCOL:%s::%c;", ctag, conf_content3.mcp_protocol);
sendtodown(sendbuf, (char)('a' + num));
}
else
{
;
}
}
//
//! ppx
//
else if(slot_type[num] == 'U')
{
if(conf_content.slot[num].ppx_mod[0] != 0xff)
{
if(strlen(conf_content.slot[num].ppx_mod) == 16)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PPX-MOD:%s::%s;", ctag, conf_content.slot[num].ppx_mod);
sendtodown(sendbuf, (char)('a' + num));
}
}
}
//
//!TOD16
//
else if(slot_type[num] == 'V')
{
if(conf_content.slot[num].tod_en[0] != 0xff)
{
if(strlen(conf_content.slot[num].tod_en) == 16)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-TOD-EN:%s::%s;", ctag, conf_content.slot[num].tod_en);
sendtodown(sendbuf, (char)('a' + num));
}
}
flag = 0;
memset(sendbuf, '\0', SENDBUFSIZE);
if(conf_content.slot[num].tod_br >= '0' && conf_content.slot[num].tod_br <= '7')
{
sprintf((char *)sendbuf, "SET-TOD-PRO:%s::%c", ctag, conf_content.slot[num].tod_br);
}
else
{
sprintf((char *)sendbuf, "SET-TOD-PRO:%s::", ctag);
}
if(conf_content.slot[num].tod_tzo >= 0x40 && conf_content.slot[num].tod_tzo <= 0x57)
{
sprintf((char *)sendbuf, "%s,%c", sendbuf, conf_content.slot[num].tod_tzo);
}
else
{
sprintf((char *)sendbuf, "%s,", sendbuf);
}
j = atoi(conf_content.slot_u.leap_num);
if((j < 1) || (j > 99))
{
if(flag == 0)
{
sprintf((char *)sendbuf, "%s,;", sendbuf);
sendtodown(sendbuf, (char)('a' + num));
}
}
else
{
sprintf((char *)sendbuf, "%s,%s;", sendbuf, conf_content.slot_u.leap_num);
sendtodown(sendbuf, (char)('a' + num));
}
}
//
//!IRIGB
//
else if(slot_type[num] == 'W')
{
if(conf_content.slot[num].igb_en[0] != 0xff)
{
if(strlen(conf_content.slot[num].igb_en) == 4)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-IGB-EN:%s::%s;", ctag, conf_content.slot[num].igb_en);
sendtodown(sendbuf, (char)('a' + num));
}
}
if(conf_content.slot[num].igb_rat[0] != 0xff)
{
if(strlen(conf_content.slot[num].igb_rat) == 4)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-IGB-RAT:%s::%s;", ctag, conf_content.slot[num].igb_rat);
sendtodown(sendbuf, (char)('a' + num));
}
}
if(conf_content.slot[num].igb_max[0] != 0xff)
{
if(strlen(conf_content.slot[num].igb_max) == 4)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-IGB-MAX:%s::%s;", ctag, conf_content.slot[num].igb_max);
sendtodown(sendbuf, (char)('a' + num));
}
}
j = atoi(conf_content.slot_u.leap_num);
if((j < 1) || (j > 99))
{
;
}
else
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-IGB-LEAP:%s::%s;", ctag, conf_content.slot_u.leap_num);
sendtodown(sendbuf, (char)('a' + num));
}
if(conf_content3.irigb_tzone[num] != 0)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-IGB-TZONE:%s::%c;", ctag, conf_content3.irigb_tzone[num]);
sendtodown(sendbuf, (char)('a' + num));
}
}
//
//REFTF
//
else if(slot_type[num] == 'S')
{
switch(conf_content.slot_u.out_ssm_oth)
{
case 0x02:
out_ssm_threshold = 'a';
break;
case 0x04:
out_ssm_threshold = 'b';
break;
case 0x08:
out_ssm_threshold = 'c';
break;
case 0x0B:
out_ssm_threshold = 'd';
break;
case 0x0F:
out_ssm_threshold = 'e';
break;
case 0x00:
out_ssm_threshold = 'f';
break;
}
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-SSM-OTH:%s::%c;", ctag, out_ssm_threshold);
sendtodown(sendbuf, (char)('a' + num));
len1 = len2 = 0;
if(conf_content.slot[num].ref_prio1[0] != 0xff)
{
len1 = strlen(conf_content.slot[num].ref_prio1);
}
if(conf_content.slot[num].ref_prio2[0] != 0xff)
{
len2 = strlen(conf_content.slot[num].ref_prio2);
}
if(len1 == 8)
{
if(len2 == 8)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-REF-PRIO:%s::%s,%s;", ctag, conf_content.slot[num].ref_prio1,
conf_content.slot[num].ref_prio2);
sendtodown(sendbuf, (char)('a' + num));
}
else
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-REF-PRIO:%s::%s,;", ctag, conf_content.slot[num].ref_prio1);
sendtodown(sendbuf, (char)('a' + num));
}
}
else
{
if(len2 == 8)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-REF-PRIO:%s::,%s;", ctag, conf_content.slot[num].ref_prio2);
sendtodown(sendbuf, (char)('a' + num));
}
}
len1 = len2 = 0;
if(conf_content.slot[num].ref_mod1[0] != 0xff)
{
len1 = strlen(conf_content.slot[num].ref_mod1);
}
if(conf_content.slot[num].ref_mod2[0] != 0xff)
{
len2 = strlen(conf_content.slot[num].ref_mod2);
}
if(len1 == 3)
{
if(len2 == 3)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-REF-MOD:%s::%s,%s;", ctag, conf_content.slot[num].ref_mod1,
conf_content.slot[num].ref_mod1);
sendtodown(sendbuf, (char)('a' + num));
}
else
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-REF-MOD:%s::%s,,;", ctag, conf_content.slot[num].ref_mod1);
sendtodown(sendbuf, (char)('a' + num));
}
}
else
{
if(len2 == 3)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-REF-MOD:%s::,,%s;", ctag, conf_content.slot[num].ref_mod2);
sendtodown(sendbuf, (char)('a' + num));
}
}
}
//
//!RS422 SF16
//
else if(slot_type[num] == 'T' || slot_type[num] == 'X')
{
if(conf_content.slot[num].rs_en[0] != 0xff)
{
if(strlen(conf_content.slot[num].rs_en) == 4)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-RS-EN:%s::%s;", ctag, conf_content.slot[num].rs_en);
sendtodown(sendbuf, (char)('a' + num));
}
}
j = atoi(conf_content.slot_u.leap_num);
if(((j < 0) || (j > 99)) && (strncmp1(conf_content.slot_u.leap_num, "00", 2) != 0))
{
if(conf_content.slot[num].rs_tzo[0] != 0xff)
{
if(strlen(conf_content.slot[num].rs_tzo) == 4)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-RS-TZO:%s::%s,;", ctag, conf_content.slot[num].rs_tzo);
sendtodown(sendbuf, (char)('a' + num));
}
}
}
else
{
if(conf_content.slot[num].rs_tzo[0] != 0xff)
{
if(strlen(conf_content.slot[num].rs_tzo) == 4)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-RS-TZO:%s::%s,%s;", ctag,
conf_content.slot[num].rs_tzo, conf_content.slot_u.leap_num);
sendtodown(sendbuf, (char)('a' + num));
return ;
}
}
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-RS-TZO:%s::,%s;", ctag, conf_content.slot_u.leap_num);
sendtodown(sendbuf, (char)('a' + num));
}
}
//
//!PTP OUT
//
else if(((slot_type[num] > 0x40) && (slot_type[num] < 0x49)) || ((slot_type[num] > 109) && (slot_type[num] < 114)))
{
j = atoi(conf_content.slot_u.leap_num);
if(strncmp1(conf_content.slot_u.leap_num, "00", 2) == 0)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PTP-LEAP:%s::%s;", ctag, conf_content.slot_u.leap_num);
sendtodown(sendbuf, (char)('a' + num));
}
else if((j < 1) || (j > 99))
{}
else
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PTP-LEAP:%s::%s;", ctag, conf_content.slot_u.leap_num);
sendtodown(sendbuf, (char)('a' + num));
}
// if((slot_type[num] == 110) || (slot_type[num] == 111)) out_port = 1;
// else if((slot_type[num] == 112) || (slot_type[num] == 113)) out_port = 2;
// else out_port = 4;
//2015.9.9 初始化ptp的协议
if( '0' == conf_content3.mcp_protocol ||
'1' == conf_content3.mcp_protocol ||
'2' == conf_content3.mcp_protocol )
{
printf("###init protocol ptp --%d\n",num);
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PROTOCOL:%s::%c;", ctag, conf_content3.mcp_protocol);
sendtodown(sendbuf, (char)('a' + num));
}
else
{
;
}
for(j = 0; j < out_port; j++)
{
if(conf_content.slot[num].ptp_type == slot_type[num])
{
/*memset(sendbuf, 0, SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PTP-MTC:%c,%d,%s;",
conf_content.slot[num].ptp_type, j + 1, conf_content3.ptp_mtc1_ip[num][j]);
printf("###run sendbuf mtc --%s\n",sendbuf);
sendtodown(sendbuf, (char)('a' + num));
usleep(200000);*/
//conf_content3.ptp_mtc1_ip[num][port]
/*MODE*/
flag = 0;
if((conf_content.slot[num].ptp_en[j] != '0') && (conf_content.slot[num].ptp_en[j] != '1'))
{
memcpy(tmp1, ",", 1);
tmp1[1] = '\0';
}
else
{
sprintf((char *)tmp1, "%c,", conf_content.slot[num].ptp_en[j]);
tmp1[2] = '\0';
flag = 1;
}
if((conf_content.slot[num].ptp_delaytype[j] != '0') && (conf_content.slot[num].ptp_delaytype[j] != '1'))
{
memcpy(tmp2, ",", 1);
tmp2[1] = '\0';
}
else
{
sprintf((char *)tmp2, "%c,", conf_content.slot[num].ptp_delaytype[j]);
tmp2[2] = '\0';
flag = 1;
}
if((conf_content.slot[num].ptp_multicast[j] != '0') && (conf_content.slot[num].ptp_multicast[j] != '1'))
{
memcpy(tmp3, ",", 1);
tmp3[1] = '\0';
}
else
{
sprintf((char *)tmp3, "%c,", conf_content.slot[num].ptp_multicast[j]);
tmp3[2] = '\0';
flag = 1;
}
if((conf_content.slot[num].ptp_enp[j] != '0') && (conf_content.slot[num].ptp_enp[j] != '1'))
{
memcpy(tmp4, ",", 1);
tmp4[1] = '\0';
}
else
{
sprintf((char *)tmp4, "%c,", conf_content.slot[num].ptp_enp[j]);
tmp4[2] = '\0';
flag = 1;
}
if((conf_content.slot[num].ptp_step[j] != '0') && (conf_content.slot[num].ptp_step[j] != '1'))
{
memcpy(tmp5, ",", 1);
tmp5[1] = '\0';
}
else
{
sprintf((char *)tmp5, "%c,", conf_content.slot[num].ptp_step[j]);
tmp5[2] = '\0';
flag = 1;
}
if(conf_content.slot[num].ptp_sync[j][0] != 0xff)
{
if(strlen(conf_content.slot[num].ptp_sync[j]) == 2)
{
flag = 1;
}
else
{
memset(conf_content.slot[num].ptp_sync[j], 0, 3);
}
}
else
{
memset(conf_content.slot[num].ptp_sync[j], 0, 3);
}
if(conf_content.slot[num].ptp_announce[j][0] != 0xff)
{
if(strlen(conf_content.slot[num].ptp_announce[j]) == 2)
{
flag = 1;
}
else
{
memset(conf_content.slot[num].ptp_announce[j], 0, 3);
}
}
else
{
memset(conf_content.slot[num].ptp_announce[j], 0, 3);
}
if(conf_content.slot[num].ptp_delayreq[j][0] != 0xff)
{
if(strlen(conf_content.slot[num].ptp_delayreq[j]) == 2)
{
flag = 1;
}
else
{
memset(conf_content.slot[num].ptp_delayreq[j], 0, 3);
}
}
else
{
memset(conf_content.slot[num].ptp_delayreq[j], 0, 3);
}
if(conf_content.slot[num].ptp_pdelayreq[j][0] != 0xff)
{
if(strlen(conf_content.slot[num].ptp_pdelayreq[j]) == 2)
{
flag = 1;
}
else
{
memset(conf_content.slot[num].ptp_pdelayreq[j], 0, 3);
}
}
else
{
memset(conf_content.slot[num].ptp_pdelayreq[j], 0, 3);
}
if(conf_content.slot[num].ptp_delaycom[j][0] != 0xff)
{
len = strlen(conf_content.slot[num].ptp_delaycom[j]);
if((len > 0) && (len < 9))
{
flag = 1;
}
else
{
memset(conf_content.slot[num].ptp_delaycom[j], 0, 9);
}
}
else
{
memset(conf_content.slot[num].ptp_delaycom[j], 0, 9);
}
if((conf_content.slot[num].ptp_linkmode[j] != '0') && (conf_content.slot[num].ptp_linkmode[j] != '1'))
{
memcpy(tmp6, ",", 1);
tmp6[1] = '\0';
tmp6[2] = '\0';
}
else
{
sprintf((char *)tmp6, "%c,", conf_content.slot[num].ptp_linkmode[j]);
tmp6[2] = '\0';
flag = 1;
}
if((conf_content.slot[num].ptp_out[j] != '0') && (conf_content.slot[num].ptp_out[j] != '1'))
{
//memcpy(tmp7, ";", 1);
memcpy(tmp7, ",", 1); //2014.12.3
tmp7[1] = '\0';
tmp7[2] = '\0';
}
else
{
sprintf((char *)tmp7, "%c,", conf_content.slot[num].ptp_out[j]); //2014.12.3
tmp7[2] = '\0';
flag = 1;
}
//2014.12.3
if(conf_content3.ptp_prio1[num][j][0] != 'A')
{
memset(&conf_content3.ptp_prio1[num][j], 0, 5);
memcpy(&conf_content3.ptp_prio1[num][j], "A128", 4);
}
else
{
;
}
if(conf_content3.ptp_prio2[num][j][0] != 'B')
{
memset(&conf_content3.ptp_prio2[num][j], 0, 5);
memcpy(&conf_content3.ptp_prio2[num][j], "B128", 4);
}
else
{
;
}
if(flag == 1)
{
//2014.12.3
//ptp配置一次低于8个选项最为安全
//为保险起见,将命令分两次发送
memset(sendbuf, '\0', SENDBUFSIZE);
//SET-PTP-MOD:%c,%d, , , , , , , , ,,,,,%s;
//<num>,<eable>,<delaytype>,<multicast>,<enp>,<step>,<sync>,<announce>,<delayreq>,<pdelayreq>,<delaycom>,<linkmode>,<outtype>,[< prio >]
sprintf((char *)sendbuf, "SET-PTP-MOD:%c,%d,,,,,,,,,,,,,%s;",
conf_content.slot[num].ptp_type,
(j + 1),
conf_content3.ptp_prio2[num][j]
);
sendtodown(sendbuf, (char)('a' + num));
usleep(200000);
memset(sendbuf, '\0', SENDBUFSIZE);
//SET-PTP-MOD:%c,%d, , , , , , , , ,,,,,%s;
//<num>,<eable>,<delaytype>,<multicast>,<enp>,<step>,<sync>,<announce>,<delayreq>,<pdelayreq>,<delaycom>,<linkmode>,<outtype>,[< prio >]
sprintf((char *)sendbuf, "SET-PTP-MOD:%c,%d,%s%s%s%s%s%s,%s,%s,%s,%s,%s%s%s;",
conf_content.slot[num].ptp_type,
(j + 1),
tmp1, tmp2, tmp3,tmp4, tmp5,
conf_content.slot[num].ptp_sync[j],
conf_content.slot[num].ptp_announce[j],
conf_content.slot[num].ptp_delayreq[j],
conf_content.slot[num].ptp_pdelayreq[j],
conf_content.slot[num].ptp_delaycom[j],
tmp6, tmp7,
conf_content3.ptp_prio1[num][j]
);/*%s%s%s,%s tmp4, tmp5,
conf_content.slot[num].ptp_sync[j],
conf_content.slot[num].ptp_announce[j],*/
//printf("ptp init send1 %s\n",sendbuf);
sendtodown(sendbuf, (char)('a' + num));
delay_s(7,0);
/*memset(sendbuf, 0, SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PTP-MOD:%c,%d,,,,,,,,%s,%s,%s,,%s;",
conf_content.slot[num].ptp_type,
(j + 1),
conf_content.slot[num].ptp_delayreq[j],
conf_content.slot[num].ptp_pdelayreq[j],
conf_content.slot[num].ptp_delaycom[j],
conf_content3.ptp_prio2[num][j]
);
//printf("ptp init send3 %s\n",sendbuf);
sendtodown(sendbuf, (char)('a' + num));
usleep(200000);
memset(sendbuf, 0, SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PTP-MOD:%c,%d,,,,,,,,,,,%s%s;",
conf_content.slot[num].ptp_type,
(j + 1),
tmp6, tmp7
);
//printf("ptp init send4 %s\n",sendbuf);
sendtodown(sendbuf, (char)('a' + num));
usleep(200000);
memset(sendbuf, '\0', SENDBUFSIZE);
//SET-PTP-MOD:%c,%d, , , , , , , , ,,,,,%s;
sprintf((char *)sendbuf, "SET-PTP-MOD:%c,%d,,,,%s%s%s,%s,,,,,,;",
conf_content.slot[num].ptp_type,
(j + 1),
tmp4, tmp5,
conf_content.slot[num].ptp_sync[j],
conf_content.slot[num].ptp_announce[j]
);
//printf("ptp init send2 %s\n",sendbuf);
sendtodown(sendbuf, (char)('a' + num));
delay_s(5,0);*/
}
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PTP-NET:%c,%d,%s,%s,%s,%s,%s,%s;",
conf_content.slot[num].ptp_type, (j + 1),
conf_content.slot[num].ptp_ip[j],
conf_content.slot[num].ptp_mac[j],
conf_content.slot[num].ptp_gate[j],
conf_content.slot[num].ptp_mask[j],
conf_content.slot[num].ptp_dns1[j],
conf_content.slot[num].ptp_dns2[j]);
//printf("###run sendbuf net --%s\n",sendbuf);
sendtodown(sendbuf, (char)('a' + num));
delay_s(3,0);
}
}
}
else if('j' == slot_type[num])
{
//
//!闰秒值下发
//
j = atoi(conf_content.slot_u.leap_num);
if(strncmp1(conf_content.slot_u.leap_num, "00", 2) == 0)
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PTP-LEAP:%s::%s;", ctag, conf_content.slot_u.leap_num);
sendtodown(sendbuf, (char)('a' + num));
}
else if((j < 1) || (j > 99))
{
;
}
else
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PTP-LEAP:%s::%s;", ctag, conf_content.slot_u.leap_num);
sendtodown(sendbuf, (char)('a' + num));
}
//
//!协议下发(联通/移动/工信)
//
if( '0' == conf_content3.mcp_protocol ||
'1' == conf_content3.mcp_protocol ||
'2' == conf_content3.mcp_protocol )
{
printf("###init protocol ptp --%d\n",num);
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PROTOCOL:%s::%c;", ctag, conf_content3.mcp_protocol);
sendtodown(sendbuf, (char)('a' + num));
printf("SET-PROTOCOL %c\n",conf_content3.mcp_protocol);
}
else
{
;
}
//
//!设置网络参数,工作模式,单播对端IP地址,光模块配置;
//
for(j = 0; j < out_port; j++)
{
if(conf_content.slot[num].ptp_type == slot_type[num])
{
//
//!Initial the net parameters。
//
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PGE4V-NET:%c,%d,,%s,%s,%s;",
conf_content.slot[num].ptp_type, (j + 1),
// conf_content.slot[num].ptp_mac[j],
conf_content.slot[num].ptp_ip[j],
conf_content.slot[num].ptp_mask[j],
conf_content.slot[num].ptp_gate[j]
);
sendtodown(sendbuf, (char)('a' + num));
usleep(200000);
//
//!Set the unicast dest parameters。
//
memset(sendbuf, 0, SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PGE4V-MTC:%c,%d,%s;",
conf_content.slot[num].ptp_type, j + 1, conf_content3.ptp_mtc_ip[num][j]);
sendtodown(sendbuf, (char)('a' + num));
usleep(200000);
//
//!Check the parameters corrected and Set Mode parameters。
//
flag = 0;
if((conf_content.slot[num].ptp_en[j] != '0') && (conf_content.slot[num].ptp_en[j] != '1'))
conf_content.slot[num].ptp_en[j] = '\0';
else
flag = 1;
if((conf_content3.ptp_esmcen[num][j] != '0') && (conf_content3.ptp_esmcen[num][j] != '1'))
conf_content3.ptp_esmcen[num][j] = '\0';
else
flag = 1;
if((conf_content.slot[num].ptp_delaytype[j] != '0') && (conf_content.slot[num].ptp_delaytype[j] != '1'))
conf_content.slot[num].ptp_delaytype[j] = '\0';
else
flag = 1;
if((conf_content.slot[num].ptp_multicast[j] != '0') && (conf_content.slot[num].ptp_multicast[j] != '1'))
conf_content.slot[num].ptp_multicast[j] = '\0';
else
flag = 1;
if((conf_content.slot[num].ptp_enp[j] != '0') && (conf_content.slot[num].ptp_enp[j] != '1') )
conf_content.slot[num].ptp_enp[j] = '\0';
else
flag = 1;
if((conf_content.slot[num].ptp_step[j] != '0') && (conf_content.slot[num].ptp_step[j] != '1'))
conf_content.slot[num].ptp_step[j] = '\0';
else
flag = 1;
//
//!Set the ptp mode。
//
if(flag == 1)
{
memset(sendbuf, 0, SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PGE4V-MOD:%c,%d,%c,%c,%c,%c,%c,%c;",
conf_content.slot[num].ptp_type, j + 1, conf_content.slot[num].ptp_en[j],
conf_content3.ptp_esmcen[num][j],
conf_content.slot[num].ptp_delaytype[j],
conf_content.slot[num].ptp_multicast[j],
conf_content.slot[num].ptp_enp[j],
conf_content.slot[num].ptp_step[j]
);
sendtodown(sendbuf, (char)('a' + num));
usleep(200000);
}
//
//!Set the ptp par。
//
flag = 0;
if((strlen(conf_content3.ptp_dom[num][j]) > 0) && (strlen(conf_content3.ptp_dom[num][j]) <= MAX_DOM_LEN))
flag = 1;
else
memset(conf_content3.ptp_dom[num][j],'\0', 4);
if(strlen(conf_content.slot[num].ptp_sync[j]) == FRAME_FRE_LEN)
flag = 1;
else
memset(conf_content.slot[num].ptp_sync[j],'\0',3);
if(strlen(conf_content.slot[num].ptp_announce[j]) == FRAME_FRE_LEN)
flag = 1;
else
memset(conf_content.slot[num].ptp_announce[j],'\0',3);
if((strlen(conf_content.slot[num].ptp_delaycom[j]) > 0) &&(strlen(conf_content.slot[num].ptp_delaycom[j]) <= DELAY_COM_LEN))
flag = 1;
else
memset(conf_content.slot[num].ptp_delaycom[j],'\0',9);
if(conf_content3.ptp_prio1[num][j][0] != 'A')
{
memset(&conf_content3.ptp_prio1[num][j], 0, 5);
memcpy(&conf_content3.ptp_prio1[num][j], "A128", 4);
}
else
{
;
}
if(conf_content3.ptp_prio2[num][j][0] != 'B')
{
memset(&conf_content3.ptp_prio2[num][j], 0, 5);
memcpy(&conf_content3.ptp_prio2[num][j], "B128", 4);
}
else
{
;
}
if(flag == 1)
{
memset(sendbuf, 0, SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PGE4V-PAR:%c,%d,%s,%s,%s,%s,%s;",
conf_content.slot[num].ptp_type, j + 1, conf_content3.ptp_dom[num][j],
conf_content.slot[num].ptp_sync[j],
conf_content.slot[num].ptp_announce[j],
conf_content.slot[num].ptp_delaycom[j],
conf_content3.ptp_prio1[num][j]
);
sendtodown(sendbuf, (char)('a' + num));
usleep(200000);
memset(sendbuf, 0, SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PGE4V-PAR:%c,%d,,,,,%s;",
conf_content.slot[num].ptp_type, j + 1, conf_content3.ptp_prio2[num][j]
);
sendtodown(sendbuf, (char)('a' + num));
usleep(200000);
}
//
//!sfp set
//
flag = 0;
if(strlen(conf_content3.ptp_sfp[num][j]) == 2)
flag = 1;
else
memset(conf_content3.ptp_sfp[num][j],'\0',3);
if((strlen(conf_content3.ptp_oplo[num][j]) > 0) && (strlen(conf_content3.ptp_oplo[num][j]) <= MAX_OPTHR_LEN))
flag = 1;
else
memset(conf_content3.ptp_oplo[num][j],'\0',6);
if((strlen(conf_content3.ptp_ophi[num][j]) > 0) && (strlen(conf_content3.ptp_ophi[num][j]) <= MAX_OPTHR_LEN))
flag = 1;
else
memset(conf_content3.ptp_ophi[num][j],'\0',6);
if(flag == 1)
{
memset(sendbuf, 0, SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PGE4V-SFP:%c,%d,%s,%s,%s;",
conf_content.slot[num].ptp_type, j + 1, conf_content3.ptp_sfp[num][j],
conf_content3.ptp_oplo[num][j],
conf_content3.ptp_ophi[num][j]
);
sendtodown(sendbuf, (char)('a' + num));
usleep(200000);
}
}
}
}
else if('s' == slot_type[num])
{
if(conf_content.slot[num].ptp_type == slot_type[num])
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PGEIN-NET:%c,%d,%s,%s,%s;",conf_content.slot[num].ptp_type, 1,
conf_content.slot[num].ptp_ip[0],
conf_content.slot[num].ptp_gate[0],
conf_content.slot[num].ptp_mask[0]);
sendtodown(sendbuf, (char)('a' + num));
usleep(200000);
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-PGEIN-MOD:%c,%d,%c,%c,%c,%c,%s,%s;",conf_content.slot[num].ptp_type,1,
conf_content.slot[num].ptp_delaytype[0],
conf_content.slot[num].ptp_multicast[0],
conf_content.slot[num].ptp_enp[0],
conf_content.slot[num].ptp_step[0],
conf_content.slot[num].ptp_delayreq[0],
conf_content.slot[num].ptp_pdelayreq[0]);
sendtodown(sendbuf, (char)('a' + num));
}
}
else if( 'Z' == slot_type[num])
{
memset(sendbuf, '\0', SENDBUFSIZE);
sprintf((char *)sendbuf, "SET-NTP-LEAP:%s::%s;", ctag, conf_content.slot_u.leap_num);
sendtodown(sendbuf, (char)('a' + num));
}
else if( 'm' == slot_type[num])
{
init_sta1(num, sendbuf);
}
}
}
int read_config3(FILE *pf)
{
if( pf == NULL )
{
return -1;
}
if(0 != fseek(pf, sizeof(file_content) + sizeof(struct extsave), SEEK_SET))
{
return 0;
}
memset(&conf_content3, 0, sizeof(file_content3));
if(1 != fread((void *)&conf_content3, sizeof(file_content3), 1, pf))
{
return 0;
}
if('T' != conf_content3.flashMask)
{
memset(&conf_content3, 0, sizeof(file_content3));
if(0 != fseek(pf, sizeof(file_content) + sizeof(struct extsave), SEEK_SET))
{
return 0;
}
fwrite((void *)&conf_content3, sizeof(file_content3), 1, pf);
fflush(pf);
sync();
system("fw -ul -f /var/p300file -o 0x1f0000 /dev/rom1");
}
else
{
}
return 1;
}
void Init_filesystem()
{
FILE *file_fd;
int rc;
//int i;
//Uint16 value;
rc = system("dd if=/dev/rom1 of=/var/p300file bs=65536 count=1 skip=31");
if(rc != 0)
{
printf("dd failed\n");
perror("system");
}
sync();
if((file_fd = fopen(FILE_DEV, "rb+")) == NULL) /*打开文件失败*/
{
printf("only read Open FILE_DEV Error:\n");
perror("fopen");
}
else
{
//printf("\nopen ok!\n");
memset(&conf_content, '\0', sizeof(file_content));
rc = fread((void *)&conf_content, sizeof(file_content), 1, file_fd);
printf("p300-0 flashmask=%c\n", conf_content.flashMask);
if('T' != conf_content.flashMask)
{
memset(&conf_content, '\0', sizeof(file_content));
if(0 != fseek(file_fd, 0, SEEK_SET))
{
printf("only SEEK_SET FILE_DEV Error:\n");
perror("SEEK_SET");
}
fwrite((void *)&conf_content, sizeof(file_content), 1, file_fd);
fflush(file_fd);
sync();
system("fw -ul -f /var/p300file -o 0x1f0000 /dev/rom1");
}
read_config3(file_fd);
fclose(file_fd);
sync();
#if 0
if((file_fd = fopen(FILE_DEV, "wb+")) < 0)
{
printf("only write open FILE_DEV Error:\n");
perror("fopen");
}
printf("\nbefor fwrite\n");
rc = fwrite((void *)&conf_content, sizeof(file_content), 1, file_fd);
fclose(file_fd);
printf("\nbefor fw\n");
system("fw -ul -f /var/p210file -o 0x1f0000 /dev/rom1");
//config_enet();
#endif
if(conf_content3.mcp_protocol <'0' ||conf_content3.mcp_protocol >'2')
conf_content3.mcp_protocol = '1';
}
}
void save_config()
{
FILE *file_fd;
if((file_fd = fopen(FILE_DEV, "rb+")) == NULL)
{
printf("Open FILE_DEV Error:\n");
}
else
{
conf_content.flashMask = 'T';
fwrite((void *)&conf_content, sizeof(file_content), 1, file_fd);
conf_content3.flashMask = 'T';
//2014.12.2
if(0 != fseek(file_fd, sizeof(file_content) + sizeof(struct extsave), SEEK_SET))
{
//return 0;
}
else
{
fwrite((void *)&conf_content3, sizeof(file_content3), 1, file_fd);
}
fflush(file_fd);
fclose(file_fd);
sync();
system("fw -ul -f /var/p300file -o 0x1f0000 /dev/rom1");
sync();
}
}
/***********************************************************************************
函数名: send_arppacket
功能: 构建arp包,以garp包告知网段内ip更新
参数: 无
返回值: 无
************************************************************************************/
void send_arppacket()
{
struct sockaddr sa;
int sock;
unsigned int ip_addr;
struct arp_packet
{
unsigned char targ_hw_addr[6];
unsigned char src_hw_addr[6];
unsigned short frame_type;
unsigned short hw_type;
unsigned short prot_type;
unsigned char hw_addr_size;
unsigned char prot_addr_size;
unsigned short op;
unsigned char sndr_hw_addr[6];
unsigned char sndr_ip_addr[4];
unsigned char rcpt_hw_addr[6];
unsigned char rcpt_ip_addr[4];
unsigned char padding[18];
} arphead;
void get_hw_addr(char *buf, char *str);
sock = socket(AF_INET, SOCK_PACKET, htons(0x0003));
arphead.frame_type = htons(0x0806);
arphead.hw_type = htons(1);
arphead.prot_type = htons(0x0800);
arphead.hw_addr_size = 6;
arphead.prot_addr_size = 4;
arphead.op = htons(1);
bzero(arphead.padding, 18);
get_hw_addr(arphead.targ_hw_addr, "ff:ff:ff:ff:ff:ff");
get_hw_addr(arphead.rcpt_hw_addr, "ff:ff:ff:ff:ff:ff");
get_hw_addr(arphead.src_hw_addr, conf_content.slot_u.mac);
get_hw_addr(arphead.sndr_hw_addr, conf_content.slot_u.mac);
ip_addr = inet_addr(conf_content.slot_u.ip);
memcpy(arphead.sndr_ip_addr, &ip_addr, 4);
memcpy(arphead.rcpt_ip_addr, &ip_addr, 4);
strcpy(sa.sa_data, "eth0");
if (sendto(sock, &arphead, sizeof(arphead), 0, &sa, sizeof(sa)) < 0)
{
perror("sendto");
return ;
}
close(sock);
printf("Garp send ok\n");
return ;
}
/***********************************************************************************
函数名: get_hw_addr
功能: 将mac地址转换为16进制
参数: char类型的mac地址
返回值: 16进制数据,存放于字节中
************************************************************************************/
void get_hw_addr(char *buf, char *str)
{
int i;
char c, val;
for (i = 0; i < 6; i++)
{
c = tolower(*str++);
if (isdigit(c))
{
val = c - '0';
}
else
{
val = c - 'a' + 10;
}
*buf = val << 4;
c = tolower(*str++);
if (isdigit(c))
{
val = c - '0';
}
else
{
val = c - 'a' + 10;
}
*buf++ |= val;
if (*str == ':')
{
str++;
}
}
}
void config_enet()
{
int len;
unsigned char mcp_ip[16];
unsigned char mcp_gateway[16];
unsigned char mcp_mask[16];
unsigned char mcp_mac[18];
memset( mcp_ip, '\0', sizeof(unsigned char) * 16);
memset( mcp_gateway, '\0', sizeof(unsigned char) * 16);
memset( mcp_mask, '\0', sizeof(unsigned char) * 16);
memset( mcp_mac, '\0', sizeof(unsigned char) * 18);
API_Get_McpIp(mcp_ip);
API_Get_McpGateWay(mcp_gateway);
len = strlen(mcp_gateway);
if(len < 7)
{
memset( mcp_gateway, '\0', sizeof(unsigned char) * 16);
}
API_Get_McpMask(mcp_mask);
API_Get_McpMacAddr(mcp_mac);
if(strncmp1(conf_content.slot_u.tid, "LT", 2) != 0)
{
memcpy(conf_content.slot_u.tid, "LT00000000000005", 16);
printf("tid=%s\n", conf_content.slot_u.tid);
//mcp_init
memcpy(conf_content.slot_u.ip, mcp_ip, strlen(mcp_ip));
memcpy(conf_content.slot_u.mask, mcp_mask, strlen(mcp_mask));
memcpy(conf_content.slot_u.gate, "192.168.1.1", 11);
API_Set_McpGateWay("192.168.1.1");
//memcpy(conf_content.slot_u.dns1,"172.16.31.10",11);
//memcpy(conf_content.slot_u.dns2,"172.16.31.10",11);
memcpy(conf_content.slot_u.mac, mcp_mac, strlen(mcp_mac));
memcpy(conf_content.slot_u.msmode, "MAIN", 4);
conf_content.slot_u.time_source = '0';
conf_content.slot_u.fb = '0';
conf_content.slot_u.out_ssm_en = '1';
conf_content.slot_u.out_ssm_oth = 0x04;
//memcpy(conf_content.slot_u.leap_num,"00",2);
}
else
{
printf("tid=%s\n", conf_content.slot_u.tid);
if(strcmp(conf_content.slot_u.ip, mcp_ip) != 0)
{
API_Set_McpIp(conf_content.slot_u.ip);
}
// if(strcmp(conf_content.slot_u.mask, mcp_mask) != 0)
{
API_Set_McpMask(conf_content.slot_u.mask);
printf("mask=%s\n", conf_content.slot_u.mask);
}
if(strcmp(conf_content.slot_u.gate, mcp_gateway) != 0)
if(if_a_string_is_a_valid_ipv4_address(conf_content.slot_u.gate) == 0)
{
API_Set_McpGateWay(conf_content.slot_u.gate);
}
if(strcmp(conf_content.slot_u.mac, mcp_mac) != 0)
{
API_Set_McpMacAddr(conf_content.slot_u.mac);
}
if((conf_content.slot_u.fb == '0') || (conf_content.slot_u.fb == '1'))
{}
else
{
conf_content.slot_u.fb = '0';
}
if( '0'!=conf_content.slot_u.time_source && '1'!=conf_content.slot_u.time_source )
{
conf_content.slot_u.time_source = '0';
}
}
#if DEBUG_NET_INFO
printf("%s:<ip>:<%s>\n", NET_INFO, conf_content.slot_u.ip);
printf("%s:<gate>:<%s>\n", NET_INFO, conf_content.slot_u.gate);
printf("%s:<mask>:<%s>\n", NET_INFO, conf_content.slot_u.mask);
printf("%s:<mac>:<%s>\n", NET_INFO, conf_content.slot_u.mac);
#endif
send_arppacket();//2014-5-19发送arp包,告知所有pc端mcp的ip与mac地址
}
int API_Set_McpIp(unsigned char *mcp_local_ip)
{
struct sockaddr_in sin;
struct ifreq ifr;
int s;
//int ret;
//unsigned char *ptr;
unsigned char *eth_name = "eth0";
memset(&ifr, '\0', sizeof(struct ifreq));
s = socket(AF_INET, SOCK_DGRAM, 0);
if(s == -1)
{
//perror("Not create network socket connection\\n");
return (-1);
}
strncpy(ifr.ifr_name, eth_name, IFNAMSIZ);
ifr.ifr_name[IFNAMSIZ - 1] = 0;
memset(&sin, '\0', sizeof(sin));
sin.sin_family = AF_INET;
sin.sin_addr.s_addr = inet_addr(mcp_local_ip);
memmove(&ifr.ifr_addr, &sin, sizeof(sin));
if(ioctl(s, SIOCSIFADDR, &ifr) < 0)
{
return (-1);
}
ifr.ifr_flags |= IFF_UP | IFF_RUNNING;
if(ioctl(s, SIOCSIFFLAGS, &ifr) < 0)
{
return (-1);
}
close(s);
return 0;
}
int API_Set_McpMask(unsigned char *mcp_local_mask)
{
int s;
unsigned char *interface_name = "eth0";
struct ifreq ifr;
struct sockaddr_in netmask_addr;
if((s = socket(PF_INET, SOCK_STREAM, 0)) < 0)
{
perror("Socket");
return (-1);
}
strcpy(ifr.ifr_name, interface_name);
memset(&netmask_addr, '\0', sizeof(struct sockaddr_in));
netmask_addr.sin_family = PF_INET;
netmask_addr.sin_addr.s_addr = inet_addr(mcp_local_mask);
memmove(&ifr.ifr_ifru.ifru_netmask, &netmask_addr, sizeof(struct sockaddr_in));
if(ioctl(s, SIOCSIFNETMASK, &ifr) < 0)
{
perror("ioctl");
return (-1);
}
close(s);
return 0;
}
int API_Set_McpGateWay(unsigned char *mcp_local_gateway)
{
unsigned char cmd[50] = "route add default gw ";
struct sockaddr_in ip_gateway;
ip_gateway.sin_addr.s_addr = inet_addr(mcp_local_gateway); //inet_addr("192.168.3.11");
system("route del default");
usleep(20);
strcat(cmd, mcp_local_gateway);
printf("%s\n", cmd);
system(cmd);
return 0;
}
int API_Set_McpMacAddr(unsigned char *mcp_local_mac)
{
unsigned char mac_add[60];
memset(mac_add, '\0', 60);
sprintf((char *)mac_add, "ifconfig eth0 hw ether %s", mcp_local_mac );
system(mac_add);
return 0;
}
int API_Get_McpIp(unsigned char *mcp_local_ip)
{
int s;
struct ifreq ifr;
struct sockaddr_in *ptr;
unsigned char *interface_name = "eth0";
if((s = socket(AF_INET, SOCK_STREAM, 0)) < 0)
{
perror("Socket");
return (-1);
}
strncpy(ifr.ifr_name, interface_name, IFNAMSIZ);
if(ioctl(s, SIOCGIFADDR, &ifr) < 0)
{
perror("Get_McuLocalIp ioctl");
return (-1);
}
ptr = (struct sockaddr_in *)&ifr.ifr_ifru.ifru_addr;
strcpy(mcp_local_ip, (unsigned char *)inet_ntoa (ptr->sin_addr)); // 获取IP地址
close(s);
//printf("get ip =%s\n",mcp_local_ip);
return 0;
}
int API_Get_McpMask(unsigned char *mcp_local_mask)
{
int s;
unsigned char *interface_name = "eth0";
struct ifreq ifr;
struct sockaddr_in *ptr;
if((s = socket(PF_INET, SOCK_STREAM, 0)) < 0)
{
perror("Socket");
return (-1);
}
strcpy(ifr.ifr_name, interface_name);
if(ioctl(s, SIOCGIFNETMASK, &ifr) < 0)
{
perror("ioctl");
return (-1);
}
ptr = (struct sockaddr_in *)&ifr.ifr_ifru.ifru_netmask;
strcpy(mcp_local_mask , (unsigned char *)inet_ntoa(ptr->sin_addr));
close(s);
//printf("get mask =%s\n",mcp_local_mask);
return 0;
}
int API_Get_McpGateWay(unsigned char *mcp_local_gateway)
{
int pl = 0, ph = 0;
int i;
FILE *rtreadfp;
unsigned char buf[1024];
memset(buf, '\0', 1024);
if((rtreadfp = popen( "route -n", "r" )) == NULL)
{
}
fread( buf, sizeof(char), sizeof(buf), rtreadfp);
pclose( rtreadfp );
for (i = 0; i < 1024; i++)
{
if (buf[i] == 'U' && buf[i + 1] == 'G' && (i + 1) < 1024)
{
pl = i;
break;
}
}
while (buf[pl] > '9' || buf[pl] < '0')
{
pl--;
}
while (('0' <= buf[pl] && buf[pl] <= '9') || buf[pl] == '.')
{
pl--;
}
while (buf[pl] > '9' || buf[pl] < '0')
{
pl--;
}
buf[pl + 1] = '\0';
for (i = pl; ('0' <= buf[i] && buf[i] <= '9') || buf[i] == '.'; i--);
ph = i + 1;
strcpy(mcp_local_gateway, &buf[ph]);
return 0;
}
int API_Get_McpMacAddr(unsigned char *mcp_local_mac)
{
int s;
struct ifreq ifr;
unsigned char *ptr;
unsigned char *interface_name = "eth0";
unsigned char buf[6];
unsigned char tmp[20];
memset(buf, '\0', 6);
memset(tmp, '\0', 20);
if((s = socket(PF_INET, SOCK_STREAM, 0)) < 0)
{
perror("Socket");
}
strcpy(ifr.ifr_name, interface_name);
if(ioctl(s, SIOCGIFHWADDR, &ifr) != 0)
{
perror("ioctl");
}
ptr = (unsigned char *)&ifr.ifr_ifru.ifru_hwaddr.sa_data[0];
buf[0] = *ptr;
buf[1] = *(ptr + 1);
buf[2] = *(ptr + 2);
buf[3] = *(ptr + 3);
buf[4] = *(ptr + 4);
buf[5] = *(ptr + 5);
sprintf((char *)tmp, "%02x:%02x:%02x:%02x:%02x:%02x",
buf[0], buf[1], buf[2], buf[3], buf[4], buf[5]);
strcpy(mcp_local_mac, tmp);
//printf("mac:%s\n",mcp_local_mac);
close(s);
return 0;
}
int if_a_string_is_a_valid_ipv4_address(const char *str)
{
struct in_addr addr;
int ret;
ret = inet_pton(AF_INET, str, &addr);
if (ret > 0)
{
return 0;
}
else
{
return (-1);
}
}
int judge_length(unsigned char *buf)
{
int len;
len = strlen_r(buf, ';') + 1;
if(len > 2)
{
if(buf[1] == (len - 2))
{
return 0;
}
else
{
return (-1);
}
}
else
{
return (-1);
}
}
int judge_client_online(int *clt_num)
{
int i, count;
count = 0;
for(i = 1; i < MAX_CLIENT; i++)
{
if(client_fd[i] != (-1))
{
clt_num[count] = i;
count++;
}
}
return count;
}
void judge_data(unsigned char *recvbuf, int len , int client)
{
unsigned char ctag[7];
unsigned char temp[20];
int ret = 0;
int len_t = 0;
memset(ctag, 0, 7);
memset(temp, 0, 20);
if(len == 10)
{
if(strncmp1("YE", recvbuf, 2) == 0)
{
memcpy(ctag, &recvbuf[3], 6);
respond_success(current_client, ctag);
}
else if(strncmp1("NO", recvbuf, 2) == 0)
{
memcpy(ctag, &recvbuf[3], 6);
respond_fail(current_client, ctag, 2);
}
else if(strncmp1("BEGINNING", recvbuf, 9) == 0)
{
process_config();
}
}
else if(strncmp1("MCP", recvbuf, 3) == 0)
{
len_t = strlen(recvbuf);
strncpy(temp, &recvbuf[8], len_t - 9);
//printf("%s\n",temp);
if(strncmp1("COM", &recvbuf[4], 3) == 0)
{
if(recvbuf[9] == '0')
{
print_switch = 0;
sendtodown("open", 'u');
}
else if(recvbuf[9] == '1')
{
print_switch = 1;
sendtodown("clse", 'u');
}
else
{
return;
}
}
else if(strncmp1("IPP", &recvbuf[4], 3) == 0)
{
if(if_a_string_is_a_valid_ipv4_address(temp) == 0)
{
ret = API_Set_McpIp(temp);
if(ret == 0)
{
memset(conf_content.slot_u.ip, '\0', 16);
memcpy(conf_content.slot_u.ip, temp, strlen(temp));
}
else
{
return;
}
}
else
{
return;
}
}
else if(strncmp1("GAT", &recvbuf[4], 3) == 0)
{
if(if_a_string_is_a_valid_ipv4_address(temp) == 0)
{
ret = API_Set_McpGateWay(temp);
if(ret == 0)
{
memset(conf_content.slot_u.gate, '\0', 16);
memcpy(conf_content.slot_u.gate, temp, strlen(temp));
}
else
{
return;
}
}
else
{
return;
}
}
else if(strncmp1("MSK", &recvbuf[4], 3) == 0)
{
if(if_a_string_is_a_valid_ipv4_address(temp) == 0)
{
ret = API_Set_McpMask(temp);
//printf("MSK=");
//printf("MSK=%s\n", temp);
if(ret == 0)
{
memset(conf_content.slot_u.mask, '\0', 16);
memcpy(conf_content.slot_u.mask, temp, strlen(temp));
}
else
{
return;
}
}
else
{
return;
}
}
else if(strncmp1("MAC", &recvbuf[4], 3) == 0)
{
if(strlen(temp) == 17)
{
ret = API_Set_McpMacAddr(temp);
if(ret == 0)
{
memset(conf_content.slot_u.mac, '\0', 18);
memcpy(conf_content.slot_u.mac, temp, 17);
}
else
{
return;
}
}
else
{
return;
}
}
else if(strncmp1("TID", &recvbuf[4], 3) == 0)
{
if(strlen(temp) == 16)
{
memset(conf_content.slot_u.tid, '\0', 18);
memcpy(conf_content.slot_u.tid, temp, 16);
}
else
{
return;
}
}
else
{
return;
}
save_config();
}
else if(strncmp1("TIME", recvbuf, 4) == 0)
{
get_time(recvbuf);
}
else if(strncmp1("FPGA", recvbuf, 4) == 0)
{
get_fpga_ver(recvbuf);
}
else if(strncmp1("R-", &recvbuf[2], 2) == 0) //判断为100s上报信息
{
if((-1) == judge_length(recvbuf))
{
printf("rpt_len error1\n");
}
else
{
process_report(recvbuf, len);
}
}
else if(strncmp1("RALM", &recvbuf[2], 4) == 0)
{
if((-1) == judge_length(recvbuf))
{
printf("rpt_len error2\n");
}
else
{
process_ralm(recvbuf, len);
}
}
else if(strncmp1("REVT", &recvbuf[2], 4) == 0)
{
if((-1) == judge_length(recvbuf))
{
printf("rpt_len error3\n");
}
else
{
process_revt(recvbuf, len);
}
}
else
{
//printf("<%s>:'recvbuf':%s\n",__FUNCTION__,recvbuf);
process_client(client, recvbuf, len); //接收到客户数据,开始处理
}
}
int judge_buff(unsigned char *buf)
{
int i;
unsigned char data[MAXDATASIZE];
unsigned char temp[20];
unsigned char passwd[9];
_MSG_NODE msg_config;
int len_data;
char slot;
memset(data, 0, MAXDATASIZE);
memset(temp, 0, 20);
memset(passwd, 0, 9);
for(i = 0; i < _CMD_NODE_MAX; i++)
{
if(strncmp1(g_cmd_fun[i].cmd , buf, g_cmd_fun[i].cmd_len) == 0)
{
//sscanf(s,"%[^:]:%[^:]:%[^;];",buf1,buf2,buf3);
sscanf(buf, "%[^:]::%c::%[^:]:%[^;];", temp, &slot, passwd, data);
memset(&msg_config, '\0', sizeof(_MSG_NODE));
//memcpy(msg_config.cmd,&recvbuf[0],i1);
//memcpy(msg_config.ctag,&recvbuf[i3],6);
len_data = strlen(data);
if(len_data > 0)
{
memcpy(msg_config.data, data, len_data);
}
else
{
return 0;
}
//msg_net.cmd_len=i1;
msg_config.solt = slot;
memcpy(msg_config.ctag, "000000", 6);
g_cmd_fun[i].cmd_fun(&msg_config, 0);
break;
}
}
return 0;
}
int process_config()
{
FILE *fp;
unsigned char buf[100];
char slot;
if((fp = fopen(CONFIG_FILE, "rt")) == NULL)
{
printf("cannot open config \n");
return 1;
}
else
{
printf(" open CONFIG \n");
}
printf("CONFIG start\n");
while(!feof(fp))
{
fgets(buf, sizeof(buf), fp);
if((buf[0] == '\r') || (buf[0] == '\0') || (buf[0] == '\n') )
{
continue;
}
else if((buf[0] == '#') || (buf[2] == '#'))
{
slot = buf[1];
}
else
{
judge_buff(buf);
/* p = buf+strlen("trap2sink ");
for(i=0; *p!='\t'&&*p!=' '; i++,p++)
str2[i]=*p;
str2[i]='\0';
*/
}
}
fclose(fp);
return 0;
}
int process_client(int client, char *recvbuf, int len)
{
unsigned char ctag[7];
unsigned char *noctag = "noctag";
int i, j, i1, i2, i3, i4, i5, len_buf;
_MSG_NODE msg_net;
memset(ctag, '\0', sizeof(unsigned char) * 7);
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
i5 = 0;
for(i = 2; i < len; i++)
{
if(recvbuf[i] == ':')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
if(j == 5)
{
i5 = i + 1;
}
}
}
if(i5 == 0)
{
return 1;
}
if((j < 5) || (recvbuf[len - 1] != ';'))
{
//出错响应
if((i4 - i3) == 6)
{
memcpy(ctag, &recvbuf[i3], i4 - i3);
}
else if((i4 - i3) == 0)
{
memcpy(ctag, noctag, 6);
}
respond_fail(client, ctag, 6);
return 1;
}
else
{
//如果tid相同,赋值到结构体(密码功能暂无)
if(strncmp1(&recvbuf[i1], conf_content.slot_u.tid, 16) == 0)
{
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:<from net>:%s\n", NET_INFO, recvbuf);
}
#endif
//printf("%s:<from net>:%s\n", NET_INFO, recvbuf);
memset(&msg_net, '\0', sizeof(_MSG_NODE));
memcpy(msg_net.cmd, &recvbuf[0], i1);
memcpy(msg_net.ctag, &recvbuf[i3], 6);
len_buf = len - i5 - 1;
if(len_buf > 0)
{
memcpy(msg_net.data, &recvbuf[i5], len_buf);
}
msg_net.cmd_len = i1;
msg_net.solt = recvbuf[i2];
cmd_process(client, &msg_net);
}
else
{
//失败响应
memcpy(ctag, &recvbuf[i3], 6);
respond_fail(client, ctag, 5);
return 1;
}
}
return 0;
}
/***********************************************************************************
函数名: event_filter
功能: 屏蔽掉多余事件
参数: 槽位号;
返回值: 无
************************************************************************************/
int event_filter(char *cmd_num)
{
char *event_id = "C23,C26,C24,C27,C28,C29,C05,C06,C07,C08,C10,C31,C42,C43,C51,C52,C61,C62,C63,C64,C67,C71,C72,C81,CB1,CB2,CB3,CC1,CC2,CC3,CC4,";
if( (NULL == cmd_num) || (cmd_num[0] != 'C') )
{
printf("event_filter para error\n");
return 0;
}
else
{
if(strstr(event_id, cmd_num) != NULL)
{
return 1;
}
else
{
return 0;
}
}
}
/***********************************************************************************
函数名: event_filter
功能: 屏蔽掉多余事件
参数: 槽位号;
返回值: 无
************************************************************************************/
int event_filter_another(char *cmd_num)
{
char *event_id = "C03,C31,C51,C52,C53,CC7,CC5,CD3,";
if( (NULL == cmd_num) || (cmd_num[0] != 'C') )
{
printf("event_filter para error\n");
return 0;
}
else
{
if(strstr(event_id, cmd_num) != NULL)
{
return 1;
}
else
{
return 0;
}
}
}
/***********************************************************************************
函数名: is_change
功能: 是否为重复上报
参数: 槽位号,上报参数
返回值: 无
************************************************************************************/
int is_change(int num, char *str)
{
int ret = 1;
int len = 0;
if(num < 0)
{
printf("is_change error pare_num %d\n", num);
return 0;
}
if(str == NULL)
{
printf("is_change error pare_str NULL\n");
return 0;
}
len = strlen(str);
ret = strncmp(event_old[num], str, len);
if(ret == 0)
{
return 0;
}
else
{
memset(event_old[num], 0, 128);
memcpy(event_old[num], str, 128);
return 1;
}
}
int process_report(unsigned char *recv, int len)
{
int i, j, i1, i2, num;
unsigned char rpt_head[10];
unsigned char rpt_data[128];
unsigned char rpt_type;
unsigned char *buf_auto = "AUTO";
//int i_temp,j_temp;
memset(rpt_head, '\0', 10);
memset(rpt_data, '\0', 128);
j = 0;
i1 = 0;
i2 = 0;
for(i = 2; i < len; i++)
{
if(recv[i] == ':')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
}
else if(recv[i] == ',')
{
j++;
if(j == 2)
{
i2 = i + 1;
}
break;
}
}
if((i1 == 0) || (i2 == 0))
{
return 1;
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:<from pan>:>%s<\n", NET_INFO, &recv[2]);
}
#endif
memcpy(rpt_head, &recv[2], i1 - 3);
memcpy(rpt_data, &recv[i2], len - i2 - 1);
rpt_type = recv[i1];
num = (int)recv[0] - 0x01;
if((num < 0) || (num > 17))
{
return 1;
}
for(i = 0; i < _NUM_RPT100S; i++)
{
if(strncmp1(RPT100S_fun[i].cmd , rpt_head , RPT100S_fun[i].cmd_len) == 0)
{
if((0 == memcmp(rpt_head, "R-OUT-CFG", 9)) || (0 == memcmp(rpt_head, "R-OUT-MGR", 9)))
{
if( (EXT_DRV != slot_type[num]) &&
((12 == num) || (13 == num)) )
{
slot_type[num] = EXT_DRV;
send_online_framing(num, EXT_DRV);
}
}
if( (EXT_DRV == slot_type[num]) &&
((12 == num) || (13 == num)) )
{
RPT100S_fun[i].rpt_fun(rpt_type, rpt_data);
}
else
{
//保存槽位类型
if(slot_type[num] != rpt_type)
{
slot_type[num] = rpt_type;
/*send 插盘事件(针对输出盘)*/
send_online_framing(num, rpt_type);
init_pan((char)(num + 'a'));
}
if(RPT100S_fun[i].cmd_num[0] == 'C')
{
if( event_filter(RPT100S_fun[i].cmd_num) )
{
//过滤不上报事件.
}
else
{
//if( is_change(num,rpt_data) )
//{
//有需要及时上报,组针上报
rpt_event_framing(RPT100S_fun[i].cmd_num, (char)(num + 'a'),
1, rpt_data, 0, buf_auto);
//}
//else
//{
//;
//}
}
}
RPT100S_fun[i].rpt_fun((char)(num + 'a'), rpt_data);
}
break;
}
}
return 0;
}
int almMsk_ExtRALM(u8_t ext_sid, unsigned char *rpt_alm,struct extctx *ctx)
{
int i,j,len;
int index = -1;
unsigned char type_flag = 0;
unsigned char num = 0;
len = strlen(rpt_alm);
if((ext_sid >= EXT_1_OUT_LOWER) && (ext_sid <= EXT_1_OUT_UPPER))
{
num = (ext_sid - EXT_1_OUT_OFFSET)%10;
type_flag = ctx->extBid[0][num];
}
else if((ext_sid >= EXT_2_OUT_LOWER) && (ext_sid <= EXT_2_OUT_UPPER))
{
num = (ext_sid - EXT_2_OUT_OFFSET)%10;
type_flag = ctx->extBid[1][num];
}
else if((ext_sid >= EXT_3_OUT_LOWER) && (ext_sid <= EXT_3_OUT_UPPER))
{
num = (ext_sid < 58) ? (ext_sid - EXT_3_OUT_OFFSET):(ext_sid - EXT_3_OUT_OFFSET - 2);//except ':;'
type_flag = ctx->extBid[2][num];
}
else if(EXT_1_MGR_PRI == ext_sid)
{
type_flag = ctx->extBid[0][10];
}
else if(EXT_1_MGR_RSV == ext_sid)
{
type_flag = ctx->extBid[0][11];
}
else if(EXT_2_MGR_PRI == ext_sid)
{
type_flag = ctx->extBid[1][10];
}
else if(EXT_2_MGR_RSV == ext_sid)
{
type_flag = ctx->extBid[1][11];
}
else if(EXT_3_MGR_PRI == ext_sid)
{
type_flag = ctx->extBid[2][10];
}
else if(EXT_3_MGR_RSV == ext_sid)
{
type_flag = ctx->extBid[2][11];
}
else
return -1;
for(j = 0; j < alm_msk_total; j++)
{
if(type_flag == conf_content.alm_msk[j].type)
{
index = j;
//printf("slot_type[num] = %c\n",slot_type[num]);
break;
}
}
if(j == alm_msk_total)
{
//printf("slottype[%d]=%c alm_msk no record\n",num,slot_type[num]);
return -1;
}
if( (rpt_alm[0] >> 4) == 0x4 && -1 != index)
{
for(i = 0; i < len; i++)
{
rpt_alm[i] &= conf_content.alm_msk[index].data[i];
}
}
return 0;
}
int almMsk_cmdRALM(int num, unsigned char *rpt_alm, int len)
{
int i, j;
int index = -1;
if(num < 0)
{
printf("%s num is error\n", __FUNCTION__);
return -1;
}
if('O' == slot_type[num])
{
return 0;
}
if(NULL == rpt_alm)
{
printf("rpt_alm is NULL\n");
return -1;
}
//20140926 drv在线告警处理
if( (12 == num || 13 == num)
&& ('d' == slot_type[num]) )
{
g_DrvLost = 200;//20141017
rpt_alm[0] = rpt_alm[0] & gExtCtx.save.onlineSta;
return 0;
}
else
{
}
for(j = 0; j < alm_msk_total; j++)
{
if(slot_type[num] == conf_content.alm_msk[j].type)
{
index = j;
//printf("slot_type[num] = %c\n",slot_type[num]);
break;
}
}
if(j == alm_msk_total)
{
//printf("slottype[%d]=%c alm_msk no record\n",num,slot_type[num]);
return -1;
}
if( (rpt_alm[0] >> 4) == 0x4 && -1 != index)
{
for(i = 0; i < len; i++)
{
rpt_alm[i] &= conf_content.alm_msk[index].data[i];
}
}
else if((rpt_alm[0] >> 4) == 0x5 && -1 != index)
{
for(i = 0; i < len; i++)
{
rpt_alm[i] &= conf_content.alm_msk[index].data[i + 6];
}
}
else
{
}
return 0;
}
/*
RB是否锁定
未锁定,对MCP中的第0位第1位 置1
*/
void is_RB_unlocked(char *rpt_alm)
{
unsigned char temp;
if(rpt_alm == NULL)
{
return ;
}
temp = rpt_alm[0];
if(slot_type[14] != 'O') //15槽位RB 盘
{
if(strncmp(rpt_content.slot_o.rb_trmode,"TRCK",4) == 0)
{
temp &= (~(1<<1));
}
else
{
temp |= (1<<1);
}
}
if(slot_type[15] != 'O') //16槽位RB 盘
{
if(strncmp(rpt_content.slot_p.rb_trmode,"TRCK",4) == 0)
{
temp &= (~(1<<0));
}
else
{
temp |= (1<<0);
}
}
rpt_alm[0] = temp;
}
//
// 改变上报的告警处理
//
int process_ralm(unsigned char *recv, int len)
{
/*<slot><len>RALM:<slotid>:<alarm>;*/
unsigned char rpt_alm[7];
unsigned char savealm[14];
unsigned char ext_alm[32];
int num;
int flag_ref = 0;
unsigned char buf[4];
memset(rpt_alm, '\0', 7);
memset(savealm, '\0', 14);
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:<from pan>:%s\n", NET_INFO, recv);
}
#endif
if(recv[7] >= 'a' && recv[7] <= 'w')
{
num = (int)(recv[7] - 'a');
if((num <= 0) || (num > 23))
{
return 1;
}
if(slot_type[num] == 'O')
{
return 1;
}
memcpy(rpt_alm, &recv[9], len - 10);
if(num == 14)
{
strncpynew(buf, rpt_content.slot_o.tod_buf, 3);
send_tod(buf, 'o');
}
else if(num == 15)
{
strncpynew(buf, rpt_content.slot_p.tod_buf, 3);
send_tod(buf, 'p');
}
else if(20 == num)
{
is_RB_unlocked(rpt_alm);
}
/*告警帧*/
//printf("<%s>\n",rpt_alm);
rpt_alm_to_client(recv[7], rpt_alm);
/*保存告警*/
/*"<aid>,<type>,<alarm>"*/
if((rpt_alm[0] >> 4) == 0x05)
{
if(slot_type[num] == 'S')
{
if((num != 1) || (num != 0))
return 1;
else
flag_ref = 1;
}
else if(slot_type[num] == 'j')
{
flag_ref = 2;
}
}
sprintf((char *)savealm, "\"%c,%c,%s\"", recv[7], slot_type[num], rpt_alm);
save_alm(recv[7], savealm, flag_ref);
//ext
if( ('m' == recv[7]) && (EXT_DRV == slot_type[12]) )
{
gExtCtx.drv[0].drvAlm = recv[9];
}
if( ('n' == recv[7]) && (EXT_DRV == slot_type[13]) )
{
gExtCtx.drv[1].drvAlm = recv[9];
}
if('u' == recv[7])
{
if(recv[13]&BIT(2))
{
gExtCtx.extMcpDrvAlm[0] = 1;
}
else
{
gExtCtx.extMcpDrvAlm[0] = 0;
}
if(recv[13]&BIT(3))
{
gExtCtx.extMcpDrvAlm[1] = 1;
}
else
{
gExtCtx.extMcpDrvAlm[1] = 0;
}
}
ext_drv2_mgr6_pr(&gExtCtx, slot_type[12], slot_type[13]);
ext_drv_mgr_evt(&gExtCtx);
}
else
{
memset(ext_alm, 0, 32);
memcpy(ext_alm, &recv[9], len - 10);
almMsk_ExtRALM(recv[7], ext_alm,&gExtCtx);
if(0 == ext_alm_cmp(recv[7], ext_alm, &gExtCtx))
{
return 1;
}
if(0 == ext_out_alm_crpt(recv[7], ext_alm, &gExtCtx))
{
return 1;
}
}
return 0;
}
int process_revt(unsigned char *recv, int len)
{
int i, j, i1, i2, i3, i4, ret;
unsigned char rpt_event[4];
unsigned char rpt_data[SENDBUFSIZE];
unsigned char rpt_reason[5];
char rpt_slot;
char tmp_buf[64];
char tp_1588_flag = 0;
memset(rpt_event, '\0', 4);
memset(rpt_data, '\0', SENDBUFSIZE);
memset(rpt_reason, '\0', 5);
j = 0;
i1 = 0;
i2 = 0;
i3 = 0;
i4 = 0;
for(i = 2; i < len; i++)
{
if(recv[i] == ':')
{
j++;
if(j == 1)
{
i1 = i + 1;
}
if(j == 2)
{
i2 = i + 1;
}
if(j == 3)
{
i3 = i + 1;
}
if(j == 4)
{
i4 = i + 1;
}
}
}
if(i4 == 0)
{
return 1;
}
rpt_slot = recv[i1];
memcpy(rpt_event, &recv[i2], 3);
memcpy(rpt_data, &recv[i3], i4 - i3 - 1);
memcpy(rpt_reason, &recv[i4], len - i4 - 1);
if((strncmp1(rpt_reason, "LMC", 3) != 0) && (strncmp1(rpt_reason, "AUTO", 4) != 0))
{
return 1;
}
//modified by zhanghui 2013-9-11 15:52:38
if('I' == slot_type[recv[0] - 1])
{
//printf("revt recv :%s\n",recv);
//printf("revt :%s\n",rpt_data);
memset(tmp_buf, 0, 64);
memcpy(tmp_buf, rpt_data, strlen(rpt_data));
tp_1588_flag = 1;
//pps state switch
if('0' == tmp_buf[0])
{
tmp_buf[0] = '1';
}
else if('1' == tmp_buf[0])
{
tmp_buf[0] = '2';
}
else if('2' == tmp_buf[0])
{
tmp_buf[0] = '3';
}
else if('5' == tmp_buf[0])
{
tmp_buf[0] = '4';
}
else
{
tmp_buf[0] = '3';
}
//source type switch
if('2' == tmp_buf[2])
{
tmp_buf[2] = '3';
}
else if('3' == tmp_buf[2])
{
tmp_buf[2] = '2';
}
else
{
//do nothing
}
}
for(i = 0; i < NUM_REVT; i++)
{
if(strncmp1(REVT_fun[i].cmd_num, rpt_event, 3) == 0)
{
//保存信息
ret = REVT_fun[i].rpt_fun(rpt_slot, rpt_data);
if(( 0 == ret ) && ( !event_filter_another(REVT_fun[i].cmd_num )))
{
if(1 == tp_1588_flag)
{
//printf("<process_revt>:%s\n",tmp_buf);
rpt_event_framing(rpt_event, (char)(recv[0] + 'a' - 1),
1, tmp_buf, 0, rpt_reason);
}
else
{
//组针上报
//rpt_event_framing(rpt_event, (char)(recv[0] + 'a' - 1),
// 1, rpt_data, 0, rpt_reason);
rpt_event_framing(rpt_event, (char)rpt_slot,
1, rpt_data, 0, rpt_reason);
}
}
break;
}
}
return 0;
}
int cmd_process(int client, _MSG_NODE *msg)
{
int i, ret;
for(i = 0; i < _CMD_NODE_MAX; i++)
{
if(strncmp1(g_cmd_fun[i].cmd, msg->cmd, g_cmd_fun[i].cmd_len) == 0)
{
current_client = client;
if(g_cmd_fun[i].cmd_fun != NULL)
{
ret = g_cmd_fun[i].cmd_fun(msg, 1);
if(ret == 0)
{
//返回成功响应
respond_success(client, msg->ctag);
return 0;
}
else
{
//返回错误响应
//printf("ret=%d\n",ret);
respond_fail(client, msg->ctag, 7);
return 0;
}
}
else
{
ret = g_cmd_fun[i].rpt_fun(client, msg);
//printf("RTRV\n");
if(ret == 0)
{
respond_success(client, msg->ctag);
}
else
{
respond_fail(client, msg->ctag, 8);
}
return 0;
}
}
}
respond_fail(client, msg->ctag, 3);
return 0;
}
int get_fpga_ver(unsigned char *data)
{
memcpy(fpga_ver, &data[5], 6);
return 0;
}
//12#drv在在没插入mge时可能不会上报告警信息,特此检查12#扩展框是否没插mge
void CheckExtLost()
{
if(slot_type[12] != 'd')
{
g_DrvLost = 200;
return;
}
else
{
if(g_DrvLost <= 0 )
{
g_DrvLost = 200;
alm_sta[12][5] = 0x4f;
alm_sta[12][5] = alm_sta[12][5] & gExtCtx.save.onlineSta;
}
else
{
g_DrvLost--;
}
}
}
void autoLeap(void)
{
static int count = 0;
_MSG_NODE *msg = NULL;
extern int cmd_set_leap_num(_MSG_NODE * msg, int flag);
if(g_LeapFlag != 1)
{
return ;
}
else
{
count++;
}
if(count >= 60)
{
msg = (_MSG_NODE *)malloc(sizeof(_MSG_NODE));
if(NULL == msg)
{
printf("autoLeap Malloc is failed\n");
return;
}
memset(msg, 0, sizeof(_MSG_NODE));
sprintf((char *)msg->cmd, "SET-LEAP-NUM:");
msg->cmd_len = 13;
msg->solt = 'u';
memcpy(msg->ctag, "R00000", 6);
memcpy(msg->data, conf_content.slot_u.leap_num, 2);
cmd_set_leap_num(msg, 1);
cmd_set_leap_num(msg, 1);
cmd_set_leap_num(msg, 1);
count = 0;
g_LeapFlag = 0;
free(msg);
}
else
{
;
}
}
int get_time(unsigned char *data) /*来自RB的时间*/
{
/*<TIME YYYY-MM-DD HH:MM:DD;>*/
int len;
unsigned char year_rb[5];
int year;
len = strlen(data);
//20141017 判断mge是否全丢失
CheckExtLost();
//20141210自动设置闰秒
if(1 == g_LeapFlag)
{
autoLeap();
}
else
{
;
}
if(len == 25)
{
if((data[9] == '-') && (data[12] == '-') && (data[18] == ':') && (data[21] == ':'))
{
memcpy(year_rb, &data[5], 4);
year = atoi(year_rb);
if(year < 2011)
{
return 1; /*如果从FPGA时间未获得,使用默认时间*/
}
memcpy(mcp_date, &data[5], 10);
memcpy(mcp_time, &data[16], 8);
}
}
else
{
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:error_time!\n", NET_INFO);
printf("%s:<%s>\n", NET_INFO, data);
}
#endif
}
return 0;
}
void get_time_fromnet()/*根据网管的配置确定时间*/
{
unsigned char year_rb[5];
unsigned char month_rb[3];
unsigned char day_rb[3];
unsigned char hour_rb[3];
unsigned char minute_rb[3];
unsigned char second_rb[3];
int year, month, day, hour, minute, second;
int tmp_hour, tmp_minute, tmp_second;
if((conf_content.slot_u.time_source == '1') && (time_temp != 0))
{
memcpy(year_rb, mcp_date, 4);
memcpy(month_rb, &mcp_date[5], 2);
memcpy(day_rb, &mcp_date[8], 2);
memcpy(hour_rb, mcp_time, 2);
memcpy(minute_rb, &mcp_time[3], 2);
memcpy(second_rb, &mcp_time[6], 2);
year = atoi(year_rb);
month = atoi(month_rb);
day = atoi(day_rb);
hour = atoi(hour_rb);
minute = atoi(minute_rb);
second = atoi(second_rb);
if(time_temp < 0)
{
tmp_hour = abs(time_temp) / 3600;
tmp_minute = (abs(time_temp) % 3600) / 60;
tmp_second = abs(time_temp) % 60;
second -= tmp_second;
minute -= tmp_minute;
hour -= tmp_hour;
if(second < 0)
{
second += 60;
minute--;
}
if(minute < 0)
{
minute += 60;
hour--;
}
if(hour < 0)
{
hour += 24;
day--;
}
if(day == 0)
{
if(month == 1)
{
day = 31;
month = 12;
year--;
}
else if((month == 2) || (month == 4) || (month == 6) || (month == 8) || (month == 9) || (month == 11))
{
day = 31;
month--;
}
else if((month == 5) || (month == 7) || (month == 10) || (month == 12))
{
day = 30;
month--;
}
else if(month == 3)
{
if(IsLeapYear(year))
{
day = 29;
}
else
{
day = 28;
}
month--;
}
}
}
else
{
tmp_hour = time_temp / 3600;
tmp_minute = (time_temp % 3600) / 60;
tmp_second = time_temp % 60;
second += tmp_second;
minute += tmp_minute;
hour += tmp_hour;
if(second > 59)
{
second = second % 60;
minute++;
}
if(minute > 59)
{
minute = minute % 60;
hour++;
}
if(hour > 23)
{
hour = hour % 24;
day++;
}
if((day == 29) && (month == 2) && (IsLeapYear(year) != 1))
{
day = 1;
month++;
}
else if((day == 30) && (month == 2) && (IsLeapYear(year) == 1))
{
day = 1;
month++;
}
else if(day == 31)
{
if((month == 4) || (month == 6) || (month == 9) || (month == 11))
{
day = 1;
month++;
}
}
else if(day == 32)
{
if((month == 1) || (month == 3) || (month == 5) || (month == 7) || (month == 8) || (month == 10))
{
day = 1;
month++;
}
else if(month == 12)
{
day = 1;
month = 1;
year++;
}
}
}
/*<TIME YYYY-MM-DD HH:MM:DD;>*/
memset(mcp_date, '\0', 11);
memset(mcp_time, '\0', 9);
sprintf((char *)mcp_date, "%04d-%02d-%02d", year, month, day);
sprintf((char *)mcp_time, "%02d:%02d:%02d", hour, minute, second);
}
}
int IsLeapYear(int year)
{
int LeapYear;
LeapYear = (((year % 4) == 0) && (year % 100 != 0)) || (year % 400 == 0);
return LeapYear;
}
void get_data(char slot, unsigned char *data)
{
int i, num;
num = (int)(slot - 'a');
sprintf((char *)data, "%s%c%c%c\"", data, SP, SP, SP);
//
//!
//
if((slot == ':') || (slot == ' '))
{
for(i = 0; i < 22; i++)
{
sprintf((char *)data, "%s%c,%c", data, (char)(0x61 + i), slot_type[i]);
if((slot_type[i] != 'O') && (i < 18))
{
if(i < 14)
{
if((EXT_DRV == slot_type[i]))
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.drv[i - 12].drvVer)) ? (char *)"," : (char *)(gExtCtx.drv[i - 12].drvVer)));
}
else
{
sprintf((char *)data, "%s,%s", data,
(strlen(rpt_content.slot[i].sy_ver) == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot[i].sy_ver));
}
}
else if(i == 14)
sprintf((char *)data, "%s,%s", data,
(strlen(rpt_content.slot_o.sy_ver) == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_o.sy_ver));
else if(i == 15)
sprintf((char *)data, "%s,%s", data,
(strlen(rpt_content.slot_p.sy_ver) == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_p.sy_ver));
else if(i == 16)
sprintf((char *)data, "%s,%s", data,
(strlen(rpt_content.slot_q.sy_ver) == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_q.sy_ver));
else if(i == 17)
sprintf((char *)data, "%s,%s", data,
(strlen(rpt_content.slot_r.sy_ver) == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_r.sy_ver));
}
else if((i == 18 )||(i == 19 ))
{
if(slot_type[i] != 'O')
sprintf((char *)data, "%s,,,,", data);
else
sprintf((char *)data, "%s", data);
}
else if(i == 20)
{
sprintf((char *)data, "%s,%s,%s,,", data, (unsigned char *)MCP_VER, fpga_ver);
}
sprintf((char *)data, "%s:", data);
}
if(slot_type[i] != 'O')
sprintf((char *)data, "%sw,%c,,,,", data, slot_type[22]);
else
sprintf((char *)data, "%sw,%c", data, slot_type[22]);
}
else if((slot >= 'a') && (slot < 'o'))
{
if(slot_type[num] == 'Y')
{
rpt_test(slot, data);
}
else if('d' == slot_type[num])
{
rpt_drv(slot, data);
}
else
{
rpt_out(slot, data);
}
}
else if((slot == 'o') || (slot == 'p'))
{
if(slot_type[num] == 'Y')
{
rpt_test(slot, data);
}
else
{
rpt_rb(slot, data);
}
}
else if((slot == 'q') || (slot == 'r'))
{
if(slot_type[num] == 'Y')
{
rpt_test(slot, data);
}
else
{
rpt_gbtp(slot, data);
}
}
else if((slot == 's') || (slot == 't'))
{
if(slot_type[num] != 'O')
sprintf((char *)data, "%s%c,%c,,,,", data, slot, slot_type[num]);
else
sprintf((char *)data, "%s%c,%c", data, slot, slot_type[num]);
}
else if(slot == 'u')
{
rpt_mcp(data);
}
else if(slot == 'v')
{
/*NULL*/
}
else if(slot == 'w')
{
if(slot_type[num] != 'O')
sprintf((char *)data, "%s%c,%c,,,,", data, slot, slot_type[num]);
else
sprintf((char *)data, "%s%c,%c", data, slot, slot_type[num]);
}
sprintf((char *)data, "%s\"%c%c;", data, CR, LF);
}
void get_ext1_eqt(char slot, unsigned char *data)
{
int i, num;
num = (int)(slot - 'a');
sprintf((char *)data, "%s%c%c%c\"", data, SP, SP, SP);
if((slot == ':') || (slot == ' '))
{
for(i = 0; i < 10; i++)
{
sprintf((char *)data, "%s%c,%c", data, (char)('a' + i), gExtCtx.extBid[0][i]);
if(gExtCtx.extBid[0][i] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.out[i].outVer)) ? (char *)(",") : (char *)(gExtCtx.out[i].outVer)));
sprintf((char *)data, "%s,%s",
data,
((0 == strlen(gExtCtx.out[i].outPR)) ? (char *)(",") : (char *)(gExtCtx.out[i].outPR)));
}
strcat(data, ":");
}
sprintf((char *)data, "%s%c,%c", data, 'k', gExtCtx.extBid[0][10]);
if(gExtCtx.extBid[0][10] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.mgr[0].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[0].mgrVer)));
}
strcat(data, ":");
sprintf((char *)data, "%s%c,%c", data, 'l', gExtCtx.extBid[0][11]);
if(gExtCtx.extBid[0][11] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.mgr[1].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[1].mgrVer)));
}
strcat(data, ":");
if(gExtCtx.extBid[0][12] == 'O')
sprintf((char *)data, "%s%c,%c", data, 'm', gExtCtx.extBid[0][12]);
else
sprintf((char *)data, "%s%c,%c,,,,", data, 'm', gExtCtx.extBid[0][12]);
strcat(data, ":");
if(gExtCtx.extBid[0][13] == 'O')
sprintf((char *)data, "%s%c,%c", data, 'n', gExtCtx.extBid[0][13]);
else
sprintf((char *)data, "%s%c,%c,,,,", data, 'n', gExtCtx.extBid[0][13]);
}
else
{
if(slot >= 'a' && slot <= 'j')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[0][slot - 'a']);
if(gExtCtx.extBid[0][slot - 'a'] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.out[slot - 'a'].outVer)) ? (char *)(",") : (char *)(gExtCtx.out[slot - 'a'].outVer)));
sprintf((char *)data, "%s,%s,%s",
data,
gExtCtx.out[slot - 'a'].outSsm,
gExtCtx.out[slot - 'a'].outSignal);
sprintf((char *)data, "%s,%s",
data,
((0 == strlen(gExtCtx.out[slot - 'a'].outPR)) ? (char *)(",") : (char *)(gExtCtx.out[slot - 'a'].outPR)));
}
}
else if(slot == 'k' || slot == 'l')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[0][slot - 'a']);
if(gExtCtx.extBid[0][slot - 'a'] != 'O')
{
sprintf((char *)data, "%s,%s,,,%d",
data,
((0 == strlen(gExtCtx.mgr[slot - 'k'].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[slot - 'k'].mgrVer)),
gExtCtx.mgr[slot - 'k'].mgrPR);
}
}
else if(slot == 'm' || slot == 'n')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[0][slot - 'a']);
}
else
{
}
}
sprintf((char *)data, "%s\"%c%c;", data, CR, LF);
}
void get_exta1_eqt(char slot, unsigned char *data)
{
int i, num;
num = (int)(slot - 'a');
sprintf((char *)data, "%s%c%c%c\"", data, SP, SP, SP);
if((slot == ':') || (slot == ' '))
{
for(i = 0; i < 10; i++)
{
sprintf((char *)data, "%s%c,%c", data, (char)('a' + i), gExtCtx.extBid[0][i]);
if(gExtCtx.extBid[0][i] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.out[i].outVer)) ? (char *)(",") : (char *)(gExtCtx.out[i].outVer)));
sprintf((char *)data, "%s,%s",
data,
((0 == strlen(gExtCtx.out[i].outPR)) ? (char *)(",") : (char *)(gExtCtx.out[i].outPR)));
}
strcat(data, ":");
}
sprintf((char *)data, "%s%c,%c", data, 'k', gExtCtx.extBid[0][10]);
if(gExtCtx.extBid[0][10] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.mgr[0].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[0].mgrVer)));
}
strcat(data, ":");
sprintf((char *)data, "%s%c,%c", data, 'l', gExtCtx.extBid[0][11]);
if(gExtCtx.extBid[0][11] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.mgr[1].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[1].mgrVer)));
}
strcat(data, ":");
if(gExtCtx.extBid[0][12] == 'O')
sprintf((char *)data, "%s%c,%c", data, 'm', gExtCtx.extBid[0][12]);
else
sprintf((char *)data, "%s%c,%c,,,,", data, 'm', gExtCtx.extBid[0][12]);
strcat(data, ":");
if(gExtCtx.extBid[0][13] == 'O')
sprintf((char *)data, "%s%c,%c", data, 'n', gExtCtx.extBid[0][13]);
else
sprintf((char *)data, "%s%c,%c,,,,", data, 'n', gExtCtx.extBid[0][13]);
}
else
{
if(slot >= 'a' && slot <= 'j')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[0][slot - 'a']);
if(gExtCtx.extBid[0][slot - 'a'] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.out[slot - 'a'].outVer)) ? (char *)(",") : (char *)(gExtCtx.out[slot - 'a'].outVer)));
sprintf((char *)data, "%s,%s,%s",
data,
gExtCtx.out[slot - 'a'].outSsm,
gExtCtx.out[slot - 'a'].outSignal);
sprintf((char *)data, "%s,%s",
data,
((0 == strlen(gExtCtx.out[slot - 'a'].outPR)) ? (char *)(",") : (char *)(gExtCtx.out[slot - 'a'].outPR)));
}
}
else if(slot == 'k' || slot == 'l')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[0][slot - 'a']);
if(gExtCtx.extBid[0][slot - 'a'] != 'O')
{
sprintf((char *)data, "%s,%s,,,%d",
data,
((0 == strlen(gExtCtx.mgr[slot - 'k'].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[slot - 'k'].mgrVer)),
gExtCtx.mgr[slot - 'k'].mgrPR);
}
}
else if(slot == 'm' || slot == 'n')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[0][slot - 'a']);
}
else
{
}
}
sprintf((char *)data, "%s\"%c%c/%c%c", data, CR, LF, CR, LF);
}
void get_ext2_eqt(char slot, unsigned char *data)
{
int i, num;
num = (int)(slot - 'a');
sprintf((char *)data, "%s%c%c%c\"", data, SP, SP, SP);
if((slot == ':') || (slot == ' '))
{
for(i = 0; i < 10; i++)
{
sprintf((char *)data, "%s%c,%c", data, (char)('a' + i), gExtCtx.extBid[1][i]);
if(gExtCtx.extBid[1][i] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.out[10 + i].outVer)) ? (char *)(",") : (char *)(gExtCtx.out[10 + i].outVer)));
sprintf((char *)data, "%s,%s",
data,
((0 == strlen(gExtCtx.out[10 + i].outPR)) ? (char *)(",") : (char *)(gExtCtx.out[10 + i].outPR)));
}
strcat(data, ":");
}
sprintf((char *)data, "%s%c,%c", data, 'k', gExtCtx.extBid[1][10]);
if(gExtCtx.extBid[1][10] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.mgr[2].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[2].mgrVer)));
}
strcat(data, ":");
sprintf((char *)data, "%s%c,%c", data, 'l', gExtCtx.extBid[1][11]);
if(gExtCtx.extBid[1][11] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.mgr[3].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[3].mgrVer)));
}
strcat(data, ":");
if(gExtCtx.extBid[1][12] == 'O')
sprintf((char *)data, "%s%c,%c", data, 'm', gExtCtx.extBid[1][12]);
else
sprintf((char *)data, "%s%c,%c,,,,", data, 'm', gExtCtx.extBid[1][12]);
strcat(data, ":");
if(gExtCtx.extBid[1][13] == 'O')
sprintf((char *)data, "%s%c,%c", data, 'n', gExtCtx.extBid[1][13]);
else
sprintf((char *)data, "%s%c,%c,,,,", data, 'n', gExtCtx.extBid[1][13]);
}
else
{
if(slot >= 'a' && slot <= 'j')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[1][slot - 'a']);
if(gExtCtx.extBid[1][slot - 'a'] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.out[10 + slot - 'a'].outVer)) ? (char *)(",") : (char *)(gExtCtx.out[10 + slot - 'a'].outVer)));
sprintf((char *)data, "%s,%s,%s",
data,
gExtCtx.out[10 + slot - 'a'].outSsm,
gExtCtx.out[10 + slot - 'a'].outSignal);
sprintf((char *)data, "%s,%s",
data,
((0 == strlen(gExtCtx.out[10 + slot - 'a'].outPR)) ? (char *)(",") : (char *)(gExtCtx.out[10 + slot - 'a'].outPR)));
}
}
else if(slot == 'k' || slot == 'l')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[1][slot - 'a']);
if(gExtCtx.extBid[1][slot - 'a'] != 'O')
{
sprintf((char *)data, "%s,%s,,,%d",
data,
((0 == strlen(gExtCtx.mgr[2 + slot - 'k'].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[2 + slot - 'k'].mgrVer)),
gExtCtx.mgr[2 + slot - 'k'].mgrPR);
}
}
else if(slot == 'm' || slot == 'n')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[1][slot - 'a']);
}
else
{
}
}
sprintf((char *)data, "%s\"%c%c;", data, CR, LF);
}
void get_exta2_eqt(char slot, unsigned char *data)
{
int i, num;
num = (int)(slot - 'a');
sprintf((char *)data, "%s%c%c%c\"", data, SP, SP, SP);
if((slot == ':') || (slot == ' '))
{
for(i = 0; i < 10; i++)
{
sprintf((char *)data, "%s%c,%c", data, (char)('a' + i), gExtCtx.extBid[1][i]);
if(gExtCtx.extBid[1][i] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.out[10 + i].outVer)) ? (char *)(",") : (char *)(gExtCtx.out[10 + i].outVer)));
sprintf((char *)data, "%s,%s",
data,
((0 == strlen(gExtCtx.out[10 + i].outPR)) ? (char *)(",") : (char *)(gExtCtx.out[10 + i].outPR)));
}
strcat(data, ":");
}
sprintf((char *)data, "%s%c,%c", data, 'k', gExtCtx.extBid[1][10]);
if(gExtCtx.extBid[1][10] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.mgr[2].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[2].mgrVer)));
}
strcat(data, ":");
sprintf((char *)data, "%s%c,%c", data, 'l', gExtCtx.extBid[1][11]);
if(gExtCtx.extBid[1][11] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.mgr[3].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[3].mgrVer)));
}
strcat(data, ":");
if(gExtCtx.extBid[1][12] == 'O')
sprintf((char *)data, "%s%c,%c", data, 'm', gExtCtx.extBid[1][12]);
else
sprintf((char *)data, "%s%c,%c,,,,", data, 'm', gExtCtx.extBid[1][12]);
strcat(data, ":");
if(gExtCtx.extBid[1][13] == 'O')
sprintf((char *)data, "%s%c,%c", data, 'n', gExtCtx.extBid[1][13]);
else
sprintf((char *)data, "%s%c,%c,,,,", data, 'n', gExtCtx.extBid[1][13]);
}
else
{
if(slot >= 'a' && slot <= 'j')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[1][slot - 'a']);
if(gExtCtx.extBid[1][slot - 'a'] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.out[10 + slot - 'a'].outVer)) ? (char *)(",") : (char *)(gExtCtx.out[10 + slot - 'a'].outVer)));
sprintf((char *)data, "%s,%s,%s",
data,
gExtCtx.out[10 + slot - 'a'].outSsm,
gExtCtx.out[10 + slot - 'a'].outSignal);
sprintf((char *)data, "%s,%s",
data,
((0 == strlen(gExtCtx.out[10 + slot - 'a'].outPR)) ? (char *)(",") : (char *)(gExtCtx.out[10 + slot - 'a'].outPR)));
}
}
else if(slot == 'k' || slot == 'l')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[1][slot - 'a']);
if(gExtCtx.extBid[1][slot - 'a'] != 'O')
{
sprintf((char *)data, "%s,%s,,,%d",
data,
((0 == strlen(gExtCtx.mgr[2 + slot - 'k'].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[2 + slot - 'k'].mgrVer)),
gExtCtx.mgr[2 + slot - 'k'].mgrPR);
}
}
else if(slot == 'm' || slot == 'n')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[1][slot - 'a']);
}
else
{
}
}
sprintf((char *)data, "%s\"%c%c/%c%c", data, CR, LF, CR, LF);
}
void get_ext3_eqt(char slot, unsigned char *data)
{
int i, num;
num = (int)(slot - 'a');
sprintf((char *)data, "%s%c%c%c\"", data, SP, SP, SP);
if((slot == ':') || (slot == ' '))
{
for(i = 0; i < 10; i++)
{
//
//! 盘类型
//
sprintf((char *)data, "%s%c,%c", data, (char)('a' + i), gExtCtx.extBid[2][i]);
//
//! 盘在线,上报
//
if(gExtCtx.extBid[2][i] != 'O')
{
//
//!版本信息
//
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.out[20 + i].outVer)) ? (char *)(",") : (char *)(gExtCtx.out[20 + i].outVer)));
//
//!模式和输出状态
//
sprintf((char *)data, "%s,%s",
data,
((0 == strlen(gExtCtx.out[20 + i].outPR)) ? (char *)(",") : (char *)(gExtCtx.out[20 + i].outPR)));
}
strcat(data, ":");
}
//
//!MGE单盘在线状态和版本
//
sprintf((char *)data, "%s%c,%c", data, 'k', gExtCtx.extBid[2][10]);
if(gExtCtx.extBid[2][10] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.mgr[4].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[4].mgrVer)));
}
strcat(data, ":");
sprintf((char *)data, "%s%c,%c", data, 'l', gExtCtx.extBid[2][11]);
if(gExtCtx.extBid[2][11] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.mgr[5].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[5].mgrVer)));
}
strcat(data, ":");
//
//!电源单盘在线状态
//
if(gExtCtx.extBid[2][12] == 'O')
sprintf((char *)data, "%s%c,%c", data, 'm', gExtCtx.extBid[2][12]);
else
sprintf((char *)data, "%s%c,%c,,,,", data, 'm', gExtCtx.extBid[2][12]);
strcat(data, ":");
if(gExtCtx.extBid[2][13] == 'O')
sprintf((char *)data, "%s%c,%c", data, 'n', gExtCtx.extBid[2][13]);
else
sprintf((char *)data, "%s%c,%c,,,,", data, 'n', gExtCtx.extBid[2][13]);
}
else
{
//
//!slot
//
if(slot >= 'a' && slot <= 'j')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[2][slot - 'a']);
if(gExtCtx.extBid[2][slot - 'a'] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.out[20 + slot - 'a'].outVer)) ? (char *)(",") : (char *)(gExtCtx.out[20 + slot - 'a'].outVer)));
sprintf((char *)data, "%s,%s,%s",
data,
gExtCtx.out[20 + slot - 'a'].outSsm,
gExtCtx.out[20 + slot - 'a'].outSignal);
sprintf((char *)data, "%s,%s",
data,
((0 == strlen(gExtCtx.out[20 + slot - 'a'].outPR)) ? (char *)(",") : (char *)(gExtCtx.out[20 + slot - 'a'].outPR)));
}
}
else if(slot == 'k' || slot == 'l')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[2][slot - 'a']);
if(gExtCtx.extBid[2][slot - 'a'] != 'O')
{
sprintf((char *)data, "%s,%s,,,%d",
data,
((0 == strlen(gExtCtx.mgr[4 + slot - 'k'].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[4 + slot - 'k'].mgrVer)),
gExtCtx.mgr[4 + slot - 'k'].mgrPR);
}
}
else if(slot == 'm' || slot == 'n')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[2][slot - 'a']);
}
else
{
}
}
sprintf((char *)data, "%s\"%c%c;", data, CR, LF);
}
void get_exta3_eqt(char slot, unsigned char *data)
{
int i, num;
num = (int)(slot - 'a');
sprintf((char *)data, "%s%c%c%c\"", data, SP, SP, SP);
if((slot == ':') || (slot == ' '))
{
for(i = 0; i < 10; i++)
{
//
//! 盘类型
//
sprintf((char *)data, "%s%c,%c", data, (char)('a' + i), gExtCtx.extBid[2][i]);
//
//! 盘在线,上报
//
if(gExtCtx.extBid[2][i] != 'O')
{
//
//!版本信息
//
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.out[20 + i].outVer)) ? (char *)(",") : (char *)(gExtCtx.out[20 + i].outVer)));
//
//!模式和输出状态
//
sprintf((char *)data, "%s,%s",
data,
((0 == strlen(gExtCtx.out[20 + i].outPR)) ? (char *)(",") : (char *)(gExtCtx.out[20 + i].outPR)));
}
strcat(data, ":");
}
//
//!MGE单盘在线状态和版本
//
sprintf((char *)data, "%s%c,%c", data, 'k', gExtCtx.extBid[2][10]);
if(gExtCtx.extBid[2][10] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.mgr[4].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[4].mgrVer)));
}
strcat(data, ":");
sprintf((char *)data, "%s%c,%c", data, 'l', gExtCtx.extBid[2][11]);
if(gExtCtx.extBid[2][11] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.mgr[5].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[5].mgrVer)));
}
strcat(data, ":");
//
//!电源单盘在线状态
//
if(gExtCtx.extBid[2][12] == 'O')
sprintf((char *)data, "%s%c,%c", data, 'm', gExtCtx.extBid[2][12]);
else
sprintf((char *)data, "%s%c,%c,,,,", data, 'm', gExtCtx.extBid[2][12]);
strcat(data, ":");
if(gExtCtx.extBid[2][13] == 'O')
sprintf((char *)data, "%s%c,%c", data, 'n', gExtCtx.extBid[2][13]);
else
sprintf((char *)data, "%s%c,%c,,,,", data, 'n', gExtCtx.extBid[2][13]);
}
else
{
//
//!slot
//
if(slot >= 'a' && slot <= 'j')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[2][slot - 'a']);
if(gExtCtx.extBid[2][slot - 'a'] != 'O')
{
sprintf((char *)data, "%s,%s,,",
data,
((0 == strlen(gExtCtx.out[20 + slot - 'a'].outVer)) ? (char *)(",") : (char *)(gExtCtx.out[20 + slot - 'a'].outVer)));
sprintf((char *)data, "%s,%s,%s",
data,
gExtCtx.out[20 + slot - 'a'].outSsm,
gExtCtx.out[20 + slot - 'a'].outSignal);
sprintf((char *)data, "%s,%s",
data,
((0 == strlen(gExtCtx.out[20 + slot - 'a'].outPR)) ? (char *)(",") : (char *)(gExtCtx.out[20 + slot - 'a'].outPR)));
}
}
else if(slot == 'k' || slot == 'l')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[2][slot - 'a']);
if(gExtCtx.extBid[2][slot - 'a'] != 'O')
{
sprintf((char *)data, "%s,%s,,,%d",
data,
((0 == strlen(gExtCtx.mgr[4 + slot - 'k'].mgrVer)) ? (char *)(",") : (char *)(gExtCtx.mgr[4 + slot - 'k'].mgrVer)),
gExtCtx.mgr[4 + slot - 'k'].mgrPR);
}
}
else if(slot == 'm' || slot == 'n')
{
sprintf((char *)data, "%s%c,%c", data, slot, gExtCtx.extBid[2][slot - 'a']);
}
else
{
}
}
sprintf((char *)data, "%s\"%c%c;", data, CR, LF);
}
void rpt_rb(char slot, unsigned char *data)
{
/*“<aid>,<type>,[<version-MCU>],[<version-FPGA>], [<version-CPLD>],[<version-PCB>],
<ref>,<priority>,<mask>,<leapmask>,<leap>,<leaptag>,
[<delay>],[<delay>],[<delay>],[<delay>],[<delay>],[<delay>],[<delay>],[<delay>],
<we>,<out>,<type-work>,<msmode>,<trmode>”*/
int i, flag_1, flag_2;
unsigned char tmp[3];
unsigned char sel_ref_mod;
memset(tmp, 0, 3);
if(slot == RB1_SLOT)
{
if(slot_type[14] == 'K' || slot_type[14] == 'R')
{
sel_ref_mod = (conf_content.slot_o.sys_ref == '0')? '0':'1';
flag_1 = strlen(rpt_content.slot_o.sy_ver);
flag_2 = strlen(rpt_content.slot_o.rf_tzo);
if(rpt_content.slot_o.rb_ref == '\0' || rpt_content.slot_o.rb_ref == 0xff)
{
tmp[0] = ',';
tmp[1] = '\0';
}
else
{
tmp[0] = rpt_content.slot_o.rb_ref;
tmp[1] = ',';
tmp[2] = '\0';
}
sprintf((char *)data, "%s%c,%c,%s,%s",
data, slot, slot_type[14], flag_1 == 0 ? (unsigned char *)COMMA_3 : rpt_content.slot_o.sy_ver,
tmp);
sprintf((char *)data, "%s%s,%s,%s,%s,1,",
data, rpt_content.slot_o.rb_prio, rpt_content.slot_o.rb_mask,
rpt_content.slot_o.rb_leapmask, rpt_content.slot_o.rb_leap
);
for(i = 0; i < 8; i++)
{
sprintf((char *)data, "%s%s,", data, rpt_content.slot_o.rf_dey[i]);
}
//20140915
if(flag_alm[14][4] == 0)
{
if('g' == rpt_content.slot_o.rb_tl_2mhz)
{
rpt_content.slot_o.rb_tl_2mhz = 'f';
}
else
{
;
}
}
else
{
rpt_content.slot_o.rb_tl_2mhz = 'g';
}
sprintf((char *)data, "%s%s,%s,%s,%s,%c,%c,%c,%c,%s,%s,%s,%s,%c,0",
data, (flag_2 == 0) ? (unsigned char *)COMMA_1 : rpt_content.slot_o.rf_tzo,
rpt_content.slot_o.rb_typework,
rpt_content.slot_o.rb_msmode,
rpt_content.slot_o.rb_trmode,
conf_content.slot_u.fb,
rpt_content.slot_o.rb_sa,
rpt_content.slot_o.rb_tl,
rpt_content.slot_o.rb_tl_2mhz,
conf_content.slot_o.thresh[0],
conf_content.slot_o.thresh[1],
rpt_content.slot_o.rb_phase[0],
rpt_content.slot_o.rb_phase[1],
sel_ref_mod
);
}
}
else if(slot == RB2_SLOT)
{
if(slot_type[15] == 'K' || slot_type[15] == 'R')
{
sel_ref_mod = (conf_content.slot_p.sys_ref == '0')? '0':'1';
/* <responsemessage>::=“<aid>,<type>,[<version-MCU>],[<version-FPGA>], [<version-CPLD>],[<version-PCB>],
<ref>,<priority>,<mask>,<leapmask>,<leap>,<leaptag>,
[<delay>],[<delay>],[<delay>],[<delay>],[<delay>],[<delay>],[<delay>],[<delay>],
<we>,<out>,<type-work>,<msmode>,<trmode>”*/
flag_1 = strlen(rpt_content.slot_p.sy_ver);
flag_2 = strlen(rpt_content.slot_p.rf_tzo);
if(rpt_content.slot_p.rb_ref == '\0' || rpt_content.slot_p.rb_ref == 0xff)
{
tmp[0] = ',';
tmp[1] = '\0';
}
else
{
tmp[0] = rpt_content.slot_p.rb_ref;
tmp[1] = ',';
tmp[2] = '\0';
}
sprintf((char *)data, "%s%c,%c,%s,%s",
data, slot, slot_type[15], (flag_1 == 0) ? (unsigned char *)COMMA_3 : rpt_content.slot_p.sy_ver,
tmp);
/* if(rpt_content.slot_p.rb_leaptag=='\0'||rpt_content.slot_p.rb_leaptag==0xff)
{
tmp[0]=',';
tmp[1]='\0';
}
else
{
tmp[0]=rpt_content.slot_o.rb_leaptag;
tmp[1]=',';
tmp[2]='\0';
}*/
sprintf((char *)data, "%s%s,%s,%s,%s,1,",
data, rpt_content.slot_p.rb_prio, rpt_content.slot_p.rb_mask,
rpt_content.slot_p.rb_leapmask, rpt_content.slot_p.rb_leap);
for(i = 0; i < 8; i++)
{
sprintf((char *)data, "%s%s,", data, rpt_content.slot_p.rf_dey[i]);
}
//20140915
if(flag_alm[15][4] == 0)
{
if('g' == rpt_content.slot_p.rb_tl_2mhz)
{
rpt_content.slot_p.rb_tl_2mhz = 'f';
}
else
{
;
}
}
else
{
rpt_content.slot_p.rb_tl_2mhz = 'g';
}
sprintf((char *)data, "%s%s,%s,%s,%s,%c,%c,%c,%c,%s,%s,%s,%s,%c,0",
data, (flag_2 == 0) ? (unsigned char *)COMMA_1 : rpt_content.slot_p.rf_tzo,
rpt_content.slot_p.rb_typework,
rpt_content.slot_p.rb_msmode,
rpt_content.slot_p.rb_trmode,
conf_content.slot_u.fb,
rpt_content.slot_p.rb_sa,
rpt_content.slot_p.rb_tl,
rpt_content.slot_p.rb_tl_2mhz,
conf_content.slot_p.thresh[0],
conf_content.slot_p.thresh[1],
rpt_content.slot_p.rb_phase[0],
rpt_content.slot_p.rb_phase[1],
sel_ref_mod);
}
}
}
void rpt_gbtp(char slot, unsigned char *data)
{
/* “<aid>,<type>,[<version-MCU>],[<version-FPGA>], [<version-CPLD>],[<version-PCB>],
<ppssta>,<sourcetype>,<pos_mode>,<elevation>, <format>, <acmode>,
<mask>, <qq-fix>,<hhmmss.ss>,<ddmm.mmmmmm>,<ns>,<dddmm.mmmmmm>,<we>,
<saaaaa.aa>,
<vvvv>,<tttttttttt>[,<sat>,<qq>,<pp>,<ss>,<h>]*”*/
int flag_1, flag_2, flag_3, flag_4, flag_5, flag_6, flag_7, flag_8, flag_9, flag_10, flag_11;
if(slot == GBTP1_SLOT)
{
if(slot_type[16] == 'L')
{
flag_1 = strlen(rpt_content.slot_q.sy_ver);
flag_2 = strlen(rpt_content.slot_q.gb_tod);
flag_3 = strlen(rpt_content.slot_q.gb_ori);
flag_4 = strlen(rpt_content.slot_q.gb_rev_g);
flag_5 = strlen(rpt_content.slot_q.gb_rev_b);
sprintf((char *) data, "%s%c,L,%s,%s,%s,%s,", data, slot,
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_q.sy_ver),
rpt_content.slot_q.bdzb,
(flag_2 == 0) ? (unsigned char *)COMMA_1 : (rpt_content.slot_q.gb_tod),
rpt_content.slot_q.gb_pos_mode);
sprintf((char *)data, "%s%s,%s,%s,", data, rpt_content.slot_q.gb_elevation,
rpt_content.slot_q.gb_format, rpt_content.slot_q.gb_acmode);
sprintf((char *)data, "%s%s,%s,%c,%s,%s", data, rpt_content.slot_q.gb_mask,
(flag_3 == 0) ? (unsigned char *)COMMA_8 : (rpt_content.slot_q.gb_ori),
conf_content.slot_u.fb,
(flag_4 == 0) ? (unsigned char *)GBTP_G : (rpt_content.slot_q.gb_rev_g),
(flag_5 == 0) ? (unsigned char *)GBTP_B : (rpt_content.slot_q.gb_rev_b));
}
else if (slot_type[16] == 'P')
{
flag_1 = strlen(rpt_content.slot_q.sy_ver);
flag_2 = strlen(rpt_content.slot_q.gb_tod);
flag_3 = strlen(rpt_content.slot_q.gb_ori);
flag_4 = strlen(rpt_content.slot_q.gb_rev_g);
sprintf((char *) data, "%s%c,P,%s,,%s,%s,", data, slot,
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_q.sy_ver),
(flag_2 == 0) ? (unsigned char *)COMMA_1 : (rpt_content.slot_q.gb_tod),
rpt_content.slot_q.gb_pos_mode);
sprintf((char *) data, "%s%s,%s,%s,", data, rpt_content.slot_q.gb_elevation,
rpt_content.slot_q.gb_format, rpt_content.slot_q.gb_acmode);
sprintf((char *)data, "%s%s,%s,%c,%s", data, rpt_content.slot_q.gb_mask,
(flag_3 == 0) ? (unsigned char *)COMMA_8 : (rpt_content.slot_q.gb_ori),
conf_content.slot_u.fb,
(flag_4 == 0) ? (unsigned char *)GBTP_G : (rpt_content.slot_q.gb_rev_g));
}
else if(slot_type[16] == 'e')
{
flag_1 = strlen(rpt_content.slot_q.sy_ver);
flag_2 = strlen(rpt_content.slot_q.gb_tod);
flag_3 = strlen(rpt_content.slot_q.gb_ori);
flag_4 = strlen(rpt_content.slot_q.gb_rev_g);
flag_5 = strlen(rpt_content.slot_q.gb_rev_b);
sprintf((char *)data, "%s%c,e,%s,%s,%s,%s,", data, slot,
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_q.sy_ver),
rpt_content.slot_q.bdzb,
(flag_2 == 0) ? (unsigned char *)COMMA_1 : (rpt_content.slot_q.gb_tod),
rpt_content.slot_q.gb_pos_mode);
sprintf((char *)data, "%s%s,%s,%s,", data, rpt_content.slot_q.gb_elevation,
rpt_content.slot_q.gb_format, rpt_content.slot_q.gb_acmode);
sprintf((char *)data, "%s%s,%s,%c,%s,%s", data, rpt_content.slot_q.gb_mask,
(flag_3 == 0) ? (unsigned char *)COMMA_8 : (rpt_content.slot_q.gb_ori),
conf_content.slot_u.fb,
(flag_4 == 0) ? (unsigned char *)GBTP_G : (rpt_content.slot_q.gb_rev_g),
(flag_5 == 0) ? (unsigned char *)GBTP_B : (rpt_content.slot_q.gb_rev_b));
}
else if(slot_type[16] == 'g')
{
flag_1 = strlen(rpt_content.slot_q.sy_ver);
flag_2 = strlen(rpt_content.slot_q.gb_tod);
flag_3 = strlen(rpt_content.slot_q.gb_ori);
flag_4 = strlen(rpt_content.slot_q.gb_rev_g);
flag_5 = strlen(rpt_content.slot_q.gb_rev_b);
sprintf((char *)data, "%s%c,g,%s,%s,%s,%s,", data, slot,
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_q.sy_ver),
rpt_content.slot_q.bdzb,
(flag_2 == 0) ? (unsigned char *)COMMA_1 : (rpt_content.slot_q.gb_tod),
rpt_content.slot_q.gb_pos_mode);
sprintf((char *)data, "%s%s,%s,%s,", data, rpt_content.slot_q.gb_elevation,
rpt_content.slot_q.gb_format, rpt_content.slot_q.gb_acmode);
sprintf((char *)data, "%s%s,%s,%c,%s,%s", data, rpt_content.slot_q.gb_mask,
(flag_3 == 0) ? (unsigned char *)COMMA_8 : (rpt_content.slot_q.gb_ori),
conf_content.slot_u.fb,
(flag_4 == 0) ? (unsigned char *)GBTP_G : (rpt_content.slot_q.gb_rev_g),
(flag_5 == 0) ? (unsigned char *)GBTP_B : (rpt_content.slot_q.gb_rev_b));
}
else if(slot_type[16] == 'l')
{
flag_1 = strlen(rpt_content.slot_q.sy_ver);
flag_2 = strlen(rpt_content.slot_q.gb_tod);
flag_3 = strlen(rpt_content.slot_q.gb_ori);
flag_4 = strlen(rpt_content.slot_q.gb_rev_g);
flag_5 = strlen(rpt_content.slot_q.gb_rev_b);
sprintf((char *)data, "%s%c,l,%s,%s,%s,%s,", data, slot,
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_q.sy_ver),
rpt_content.slot_q.bdzb,
(flag_2 == 0) ? (unsigned char *)COMMA_1 : (rpt_content.slot_q.gb_tod),
rpt_content.slot_q.gb_pos_mode);
sprintf((char *)data, "%s%s,%s,%s,", data, rpt_content.slot_q.gb_elevation,
rpt_content.slot_q.gb_format, rpt_content.slot_q.gb_acmode);
sprintf((char *)data, "%s%s,%s,%c,%s,%s", data, rpt_content.slot_q.gb_mask,
(flag_3 == 0) ? (unsigned char *)COMMA_8 : (rpt_content.slot_q.gb_ori),
conf_content.slot_u.fb,
(flag_4 == 0) ? (unsigned char *)GBTP_G : (rpt_content.slot_q.gb_rev_g),
(flag_5 == 0) ? (unsigned char *)GBTP_B : (rpt_content.slot_q.gb_rev_b));
}
else if(slot_type[16] == 'v')
{
flag_1 = strlen(rpt_content.slot_q.sy_ver);
flag_2 = (rpt_content.slot_q.gb_sta == '\0') ? 0:1;
flag_3 = (rpt_content.slot_q.staen == '\0') ? 0:1;
//flag_4 = strlen(rpt_content.slot_q.gb_pos_mode);
//flag_5 = strlen(rpt_content.slot_q.gb_acmode);
flag_6 = rpt_content.slot_q.gb_tod[0] == '\0' ? 0:1;
flag_7 = strlen(rpt_content.slot_q.leapalm);
flag_8 = strlen(rpt_content.slot_q.leapnum);
flag_9 = strlen(rpt_content.slot_q.gb_ori);
flag_10 = strlen(rpt_content.slot_q.gb_rev_g);
flag_11 = strlen(rpt_content.slot_q.gb_rev_b);
sprintf((char *)data, "%s%c,v,%s,%c,%c,%s,%s,%c,%s,%s,", data, slot,
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_q.sy_ver),
(flag_2 == 0) ? '2' : rpt_content.slot_q.gb_sta,
(flag_3 == 0) ? '1' : rpt_content.slot_q.staen,
rpt_content.slot_q.gb_pos_mode,
rpt_content.slot_q.gb_acmode,
(flag_6 == 0) ? '3' : rpt_content.slot_q.gb_tod[0],
(flag_7 == 0) ? "00": (char *)rpt_content.slot_q.leapalm,
(flag_8 == 0) ? "00": (char *)rpt_content.slot_q.leapnum);
sprintf((char *)data, "%s%s,%s,%s,%s,%s", data,
((flag_9 == 0) ? (unsigned char *)COMMA_9 : rpt_content.slot_q.gb_ori),
rpt_content.slot_q.rec_type,
rpt_content.slot_q.rec_ver,
((flag_10 == 0) ? (unsigned char *)GBTP_GPS : rpt_content.slot_q.gb_rev_g),
((flag_11 == 0) ? (unsigned char *)GBTP_BD : rpt_content.slot_q.gb_rev_b));
}
else if(slot_type[16] == 'x')
{
flag_1 = strlen(rpt_content.slot_q.sy_ver);
flag_2 = (rpt_content.slot_q.gb_sta == '\0') ? 0:1;
flag_3 = (rpt_content.slot_q.staen == '\0') ? 0:1;
//flag_4 = strlen(rpt_content.slot_q.gb_pos_mode);
//flag_5 = strlen(rpt_content.slot_q.gb_acmode);
//flag_4 = strlen(rpt_content.slot_q.delay);
flag_6 = rpt_content.slot_q.gb_tod[0] == '\0' ? 0:1;
flag_7 = strlen(rpt_content.slot_q.leapalm);
flag_8 = strlen(rpt_content.slot_q.leapnum);
flag_9 = strlen(rpt_content.slot_q.gb_ori);
flag_10 = strlen(rpt_content.slot_q.gb_rev_g);
flag_11 = strlen(rpt_content.slot_q.gb_rev_b);
flag_4 = strlen(rpt_content.slot_q.gb_rev_glo);
flag_5 = strlen(rpt_content.slot_q.gb_rev_gal);
sprintf((char *)data, "%s%c,x,%s,%c,%c,%s,%s,%s,%s,%c,%s,%s,", data, slot,
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_q.sy_ver),
(flag_2 == 0) ? '2' : rpt_content.slot_q.gb_sta,
(flag_3 == 0) ? '1' : rpt_content.slot_q.staen,
rpt_content.slot_q.gb_pos_mode,
rpt_content.slot_q.gb_acmode,
rpt_content.slot_q.delay,
rpt_content.slot_q.pps_out_delay,
(flag_6 == 0) ? '3' : rpt_content.slot_q.gb_tod[0],
(flag_7 == 0) ? "00": (char *)rpt_content.slot_q.leapalm,
(flag_8 == 0) ? "00": (char *)rpt_content.slot_q.leapnum);
sprintf((char *)data, "%s%s,%s,%s,%s,%s,%s,%s", data,
((flag_9 == 0) ? (unsigned char *)COMMA_9 : rpt_content.slot_q.gb_ori),
rpt_content.slot_q.rec_type,
rpt_content.slot_q.rec_ver,
((flag_10 == 0) ? (unsigned char *)GBTP_GPS : rpt_content.slot_q.gb_rev_g),
((flag_11 == 0) ? (unsigned char *)GBTP_BD : rpt_content.slot_q.gb_rev_b),
((flag_4 == 0) ? (unsigned char *)GBTP_GLO : rpt_content.slot_q.gb_rev_glo),
((flag_5 == 0) ? (unsigned char *)GBTP_GAL : rpt_content.slot_q.gb_rev_gal));
}
}
else if(slot == GBTP2_SLOT)
{
if(slot_type[17] == 'L')
{
flag_1 = strlen(rpt_content.slot_r.sy_ver);
flag_2 = strlen(rpt_content.slot_r.gb_tod);
flag_3 = strlen(rpt_content.slot_r.gb_ori);
flag_4 = strlen(rpt_content.slot_r.gb_rev_g);
flag_5 = strlen(rpt_content.slot_r.gb_rev_b);
sprintf((char *)data, "%s%c,L,%s,%s,%s,%s,", data, slot,
flag_1 == 0 ? (unsigned char *)COMMA_3 : (rpt_content.slot_r.sy_ver),
rpt_content.slot_r.bdzb,
flag_2 == 0 ? (unsigned char *)COMMA_1 : (rpt_content.slot_r.gb_tod),
rpt_content.slot_r.gb_pos_mode);
sprintf((char *)data, "%s%s,%s,%s,", data, rpt_content.slot_r.gb_elevation,
rpt_content.slot_r.gb_format, rpt_content.slot_r.gb_acmode);
sprintf((char *)data, "%s%s,%s,%c,%s,%s", data, rpt_content.slot_r.gb_mask,
flag_3 == 0 ? (unsigned char *)COMMA_8 : (rpt_content.slot_r.gb_ori),
conf_content.slot_u.fb,
flag_4 == 0 ? (unsigned char *)GBTP_G : (rpt_content.slot_r.gb_rev_g),
flag_5 == 0 ? (unsigned char *)GBTP_B : (rpt_content.slot_r.gb_rev_b));
}
else if(slot_type[17] == 'P')
{
flag_1 = strlen(rpt_content.slot_r.sy_ver);
flag_2 = strlen(rpt_content.slot_r.gb_tod);
flag_3 = strlen(rpt_content.slot_r.gb_ori);
flag_4 = strlen(rpt_content.slot_r.gb_rev_g);
sprintf((char *)data, "%s%c,P,%s,,%s,%s,", data, slot,
flag_1 == 0 ? (unsigned char *)COMMA_3 : (rpt_content.slot_r.sy_ver),
flag_2 == 0 ? (unsigned char *)COMMA_1 : (rpt_content.slot_r.gb_tod),
rpt_content.slot_r.gb_pos_mode);
sprintf((char *)data, "%s%s,%s,%s,", data, rpt_content.slot_r.gb_elevation,
rpt_content.slot_r.gb_format, rpt_content.slot_r.gb_acmode);
sprintf((char *)data, "%s%s,%s,%c,%s", data, rpt_content.slot_r.gb_mask,
flag_3 == 0 ? (unsigned char *)COMMA_8 : (rpt_content.slot_r.gb_ori),
conf_content.slot_u.fb,
flag_4 == 0 ? (unsigned char *)GBTP_G : (rpt_content.slot_r.gb_rev_g));
}
else if(slot_type[17] == 'e')
{
flag_1 = strlen(rpt_content.slot_r.sy_ver);
flag_2 = strlen(rpt_content.slot_r.gb_tod);
flag_3 = strlen(rpt_content.slot_r.gb_ori);
flag_4 = strlen(rpt_content.slot_r.gb_rev_g);
flag_5 = strlen(rpt_content.slot_r.gb_rev_b);
sprintf((char *)data, "%s%c,e,%s,%s,%s,%s,", data, slot,
flag_1 == 0 ? (unsigned char *)COMMA_3 : (rpt_content.slot_r.sy_ver),
rpt_content.slot_r.bdzb,
flag_2 == 0 ? (unsigned char *)COMMA_1 : (rpt_content.slot_r.gb_tod),
rpt_content.slot_r.gb_pos_mode);
sprintf((char *)data, "%s%s,%s,%s,", data, rpt_content.slot_r.gb_elevation,
rpt_content.slot_r.gb_format, rpt_content.slot_r.gb_acmode);
sprintf((char *)data, "%s%s,%s,%c,%s,%s", data, rpt_content.slot_r.gb_mask,
flag_3 == 0 ? (unsigned char *)COMMA_8 : (rpt_content.slot_r.gb_ori),
conf_content.slot_u.fb,
flag_4 == 0 ? (unsigned char *)GBTP_G : (rpt_content.slot_r.gb_rev_g),
flag_5 == 0 ? (unsigned char *)GBTP_B : (rpt_content.slot_r.gb_rev_b));
}
else if(slot_type[17] == 'g')
{
flag_1 = strlen(rpt_content.slot_r.sy_ver);
flag_2 = strlen(rpt_content.slot_r.gb_tod);
flag_3 = strlen(rpt_content.slot_r.gb_ori);
flag_4 = strlen(rpt_content.slot_r.gb_rev_g);
flag_5 = strlen(rpt_content.slot_r.gb_rev_b);
sprintf((char *)data, "%s%c,g,%s,%s,%s,%s,", data, slot,
flag_1 == 0 ? (unsigned char *)COMMA_3 : (rpt_content.slot_r.sy_ver),
rpt_content.slot_r.bdzb,
flag_2 == 0 ? (unsigned char *)COMMA_1 : (rpt_content.slot_r.gb_tod),
rpt_content.slot_r.gb_pos_mode);
sprintf((char *)data, "%s%s,%s,%s,", data, rpt_content.slot_r.gb_elevation,
rpt_content.slot_r.gb_format, rpt_content.slot_r.gb_acmode);
sprintf((char *)data, "%s%s,%s,%c,%s,%s", data, rpt_content.slot_r.gb_mask,
flag_3 == 0 ? (unsigned char *)COMMA_8 : (rpt_content.slot_r.gb_ori),
conf_content.slot_u.fb,
flag_4 == 0 ? (unsigned char *)GBTP_G : (rpt_content.slot_r.gb_rev_g),
flag_5 == 0 ? (unsigned char *)GBTP_B : (rpt_content.slot_r.gb_rev_b));
}
else if(slot_type[17] == 'l')
{
flag_1 = strlen(rpt_content.slot_r.sy_ver);
flag_2 = strlen(rpt_content.slot_r.gb_tod);
flag_3 = strlen(rpt_content.slot_r.gb_ori);
flag_4 = strlen(rpt_content.slot_r.gb_rev_g);
flag_5 = strlen(rpt_content.slot_r.gb_rev_b);
sprintf((char *)data, "%s%c,l,%s,%s,%s,%s,", data, slot,
flag_1 == 0 ? (unsigned char *)COMMA_3 : (rpt_content.slot_r.sy_ver),
rpt_content.slot_r.bdzb,
flag_2 == 0 ? (unsigned char *)COMMA_1 : (rpt_content.slot_r.gb_tod),
rpt_content.slot_r.gb_pos_mode);
sprintf((char *)data, "%s%s,%s,%s,", data, rpt_content.slot_r.gb_elevation,
rpt_content.slot_r.gb_format, rpt_content.slot_r.gb_acmode);
sprintf((char *)data, "%s%s,%s,%c,%s,%s", data, rpt_content.slot_r.gb_mask,
flag_3 == 0 ? (unsigned char *)COMMA_8 : (rpt_content.slot_r.gb_ori),
conf_content.slot_u.fb,
flag_4 == 0 ? (unsigned char *)GBTP_G : (rpt_content.slot_r.gb_rev_g),
flag_5 == 0 ? (unsigned char *)GBTP_B : (rpt_content.slot_r.gb_rev_b));
}
else if(slot_type[17] == 'v')
{
flag_1 = strlen(rpt_content.slot_r.sy_ver);
flag_2 = (rpt_content.slot_r.gb_sta == '\0') ? 0:1;
flag_3 = (rpt_content.slot_r.staen == '\0') ? 0:1;
//flag_4 = strlen(rpt_content.slot_q.gb_pos_mode);
//flag_5 = strlen(rpt_content.slot_q.gb_acmode);
flag_6 = rpt_content.slot_r.gb_tod[0] == '\0' ? 0:1;
flag_7 = strlen(rpt_content.slot_r.leapalm);
flag_8 = strlen(rpt_content.slot_r.leapnum);
flag_9 = strlen(rpt_content.slot_r.gb_ori);
flag_10 = strlen(rpt_content.slot_r.gb_rev_g);
flag_11 = strlen(rpt_content.slot_r.gb_rev_b);
sprintf((char *)data, "%s%c,v,%s,%c,%c,%s,%s,%c,%s,%s,", data, slot,
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_r.sy_ver),
(flag_2 == 0) ? '2' : rpt_content.slot_r.gb_sta,
(flag_3 == 0) ? '1' : rpt_content.slot_r.staen,
rpt_content.slot_r.gb_pos_mode,
rpt_content.slot_r.gb_acmode,
(flag_6 == 0) ? '3' : rpt_content.slot_r.gb_tod[0],
(flag_7 == 0) ? "00": (char *)rpt_content.slot_r.leapalm,
(flag_8 == 0) ? "00": (char *)rpt_content.slot_r.leapnum);
sprintf((char *)data, "%s%s,%s,%s,%s,%s", data,
((flag_9 == 0) ? (unsigned char *)COMMA_9 : rpt_content.slot_r.gb_ori),
rpt_content.slot_r.rec_type,
rpt_content.slot_r.rec_ver,
((flag_10 == 0) ? (unsigned char *)GBTP_GPS : rpt_content.slot_r.gb_rev_g),
((flag_11 == 0) ? (unsigned char *)GBTP_BD : rpt_content.slot_r.gb_rev_b));
}
else if(slot_type[17] == 'x')
{
flag_1 = strlen(rpt_content.slot_r.sy_ver);
flag_2 = (rpt_content.slot_r.gb_sta == '\0') ? 0:1;
flag_3 = (rpt_content.slot_r.staen == '\0') ? 0:1;
//flag_4 = strlen(rpt_content.slot_q.gb_pos_mode);
//flag_5 = strlen(rpt_content.slot_q.gb_acmode);
//flag_4 = strlen(rpt_content.slot_q.delay);
flag_6 = rpt_content.slot_r.gb_tod[0] == '\0' ? 0:1;
flag_7 = strlen(rpt_content.slot_r.leapalm);
flag_8 = strlen(rpt_content.slot_r.leapnum);
flag_9 = strlen(rpt_content.slot_r.gb_ori);
flag_10 = strlen(rpt_content.slot_r.gb_rev_g);
flag_11 = strlen(rpt_content.slot_r.gb_rev_b);
flag_4 = strlen(rpt_content.slot_r.gb_rev_glo);
flag_5 = strlen(rpt_content.slot_r.gb_rev_gal);
sprintf((char *)data, "%s%c,x,%s,%c,%c,%s,%s,%s,%s,%c,%s,%s,", data, slot,
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot_r.sy_ver),
(flag_2 == 0) ? '2' : rpt_content.slot_r.gb_sta,
(flag_3 == 0) ? '1' : rpt_content.slot_r.staen,
rpt_content.slot_r.gb_pos_mode,
rpt_content.slot_r.gb_acmode,
rpt_content.slot_r.delay,
rpt_content.slot_r.pps_out_delay,
(flag_6 == 0) ? '3' : rpt_content.slot_r.gb_tod[0],
(flag_7 == 0) ? "00": (char *)rpt_content.slot_r.leapalm,
(flag_8 == 0) ? "00": (char *)rpt_content.slot_r.leapnum);
sprintf((char *)data, "%s%s,%s,%s,%s,%s,%s,%s", data,
((flag_9 == 0) ? (unsigned char *)COMMA_9 : rpt_content.slot_r.gb_ori),
rpt_content.slot_r.rec_type,
rpt_content.slot_r.rec_ver,
((flag_10 == 0) ? (unsigned char *)GBTP_GPS : rpt_content.slot_r.gb_rev_g),
((flag_11 == 0) ? (unsigned char *)GBTP_BD : rpt_content.slot_r.gb_rev_b),
((flag_4 == 0) ? (unsigned char *)GBTP_GLO : rpt_content.slot_r.gb_rev_glo),
((flag_5 == 0) ? (unsigned char *)GBTP_GAL : rpt_content.slot_r.gb_rev_gal));
}
}
}
void get_sta1_ver(unsigned char *data, int num)
{
//sprintf((char *)data,"%s,%s,%s,,1,JLABAE@C,JLABAE@A,OOOOOO@@,,,",data,slot,"V01.00","V01.00");
if(NULL != strchr(rpt_content.slot[num].sy_ver,','))
{
sprintf((char *)data, "%s,%s1", data, rpt_content.slot[num].sy_ver);
}
else
{
sprintf((char *)data, "%s,,,,1", data);
}
if( (( (conf_content3.sta1.ip[0] ) & 0xf0 ) != 0x40) ||
(((conf_content3.sta1.ip[2] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.ip[4] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.ip[6] ) & 0xf0) != 0x40) )
{
memset(conf_content3.sta1.ip, 0, 9);
memcpy(conf_content3.sta1.ip, "L@JH@AFC", 8);
}
if( (((conf_content3.sta1.gate[0] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.gate[2] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.gate[4] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.gate[6] ) & 0xf0) != 0x40) )
{
memset(conf_content3.sta1.gate, 0, 9);
memcpy(conf_content3.sta1.gate, "L@JH@A@A", 8);
}
if( (((conf_content3.sta1.mask[0] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.mask[2] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.mask[4] ) & 0xf0) != 0x40) ||
(((conf_content3.sta1.mask[6] ) & 0xf0) != 0x40) )
{
memset(conf_content3.sta1.mask, 0, 9);
memcpy(conf_content3.sta1.mask, "OOOOOO@@", 8);
}
sprintf((char *)data, "%s,%s,%s,%s,,,", data, conf_content3.sta1.ip, conf_content3.sta1.gate, conf_content3.sta1.mask);
//sprintf((char *)data,"%s,%s,%s,,1,JLABAE@C,JLABAE@A,OOOOOO@@,,,",data,slot,"V01.00","V01.00");
}
void __srtcat(unsigned char *dist,unsigned char *tail)
{
int res;
unsigned char comma[2];
comma[0] = ',';
comma[1] = 0;
if(dist == NULL || tail == NULL)
{
return ;
}
strcat(dist,comma);
res = strlen(tail);
if(0 == res)
{
//strcat(dist,",");
}
else
{
strcat(dist,tail);
}
}
void str_2_net(unsigned char net_addr,unsigned char *h_buf,unsigned char *l_buf)
{
unsigned char h_4bit,l_4bit;
l_4bit = (net_addr & 0x0f) | 0x40;
h_4bit = ((net_addr & 0xf0) >> 4) | 0x40;
//printf("o:%x\n",net_addr);
//printf("h:%x,l:%x\n",h_4bit,l_4bit);
*h_buf = h_4bit;
*l_buf = l_4bit;
}
void strcat_mac(unsigned char * data,int num,int port)
{
int i;
char buf[13];
memset(buf,0,13);
for(i=0;i<6;i++)
{
str_2_net(rpt_content.slot[num].ntp_pmac[port][i],&buf[i*2],&buf[i*2+1]);
}
__srtcat(data,buf);
}
void strcat_ip(unsigned char * data,int num,int port)
{
int i;
char buf[13];
memset(buf,0,13);
for(i=0;i<4;i++)
{
str_2_net(rpt_content.slot[num].ntp_pip[port][i],&buf[i*2],&buf[i*2+1]);
}
__srtcat(data,buf);
}
void strcat_gate(unsigned char * data,int num,int port)
{
int i;
char buf[13];
memset(buf,0,13);
for(i=0;i<4;i++)
{
str_2_net(rpt_content.slot[num].ntp_pgateway[port][i],&buf[i*2],&buf[i*2+1]);
}
__srtcat(data,buf);
}
void strcat_mask(unsigned char * data,int num,int port)
{
int i;
char buf[13];
memset(buf,0,13);
for(i=0;i<4;i++)
{
str_2_net(rpt_content.slot[num].ntp_pnetmask[port][i],&buf[i*2],&buf[i*2+1]);
}
__srtcat(data,buf);
}
void rpt_out(char slot, unsigned char *data)
{
int num, i;
unsigned char tmp[4];
unsigned char tmp1[3], tmp2[3], tmp3[3], tmp4[3];
int flag_1, flag_2;
unsigned char tmp_buf[4];
int length = 0;
num = (int)(slot - 'a');
if(slot_type[num] == 'I')
{
/* "<aid>,<type>,
[<version-MCU>],[<version-FPGA>], [<version-CPLD>],[<version-PCB>],
<ppssta>,<sourcetype>,<en>”*/
flag_1 = strlen(rpt_content.slot[num].sy_ver);
flag_2 = strlen(rpt_content.slot[num].tp16_tod);
memcpy(tmp_buf, rpt_content.slot[num].tp16_tod, strlen(rpt_content.slot[num].tp16_tod));
//
//pps state switch
//
if('0' == tmp_buf[0])
{
tmp_buf[0] = '1';
}
else if('1' == tmp_buf[0])
{
tmp_buf[0] = '2';
}
else if('2' == tmp_buf[0])
{
tmp_buf[0] = '3';
}
else if('5' == tmp_buf[0])
{
tmp_buf[0] = '4';
}
else
{
tmp_buf[0] = '3';
}
//source type switch
if('2' == tmp_buf[2])
{
tmp_buf[2] = '3';
}
else if('3' == tmp_buf[2])
{
tmp_buf[2] = '2';
}
else
{
//do nothing
}
//printf("%s\t%s\n",rpt_content.slot[num].tp16_tod,tmp_buf);
sprintf((char *)data, "%s%c,I,%s,%s,%s", data, slot,
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot[num].sy_ver),
(flag_2 == 0) ? (unsigned char *)COMMA_1 : tmp_buf,
rpt_content.slot[num].tp16_en);
}
else if( (slot_type[num] == 'J') ||
(slot_type[num] == 'a') ||
(slot_type[num] == 'b') ||
(slot_type[num] == 'c') ||
(slot_type[num] == 'h') ||
(slot_type[num] == 'i'))
{
if((rpt_content.slot[num].out_mode == '0') || (rpt_content.slot[num].out_mode == '1'))
{}
else
{
rpt_content.slot[num].out_mode = '0';
}
flag_1 = strlen(rpt_content.slot[num].sy_ver);
sprintf((char *)data, "%s%c,%c,%s,%s,%s,%c,%c", data, slot, slot_type[num],
flag_1 == 0 ? (unsigned char *)(COMMA_3) : (rpt_content.slot[num].sy_ver),
rpt_content.slot[num].out_lev,
rpt_content.slot[num].out_typ,
rpt_content.slot[num].out_mode,
rpt_content.slot[num].out_sta);
}
else if(((slot_type[num] > '@') && (slot_type[num] < 'I')) || ((slot_type[num] > 109) && (slot_type[num] < 114)) )//PTP
{
flag_1 = strlen(rpt_content.slot[num].sy_ver);
sprintf((char *)data, "%s%c,%c,%s", data, slot, slot_type[num],
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot[num].sy_ver));
for(i = 0; i < 4; i++)
{
sprintf((char *)data, "%s,%d,%s,%s,%s,%s,%s,%s", data, i + 1,
rpt_content.slot[num].ptp_ip[i],
rpt_content.slot[num].ptp_mac[i],
rpt_content.slot[num].ptp_gate[i],
rpt_content.slot[num].ptp_mask[i],
rpt_content.slot[num].ptp_dns1[i],
rpt_content.slot[num].ptp_dns2[i]);
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_en[i]);
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_delaytype[i]);
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_multicast[i]);
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_enp[i]);
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_step[i]);
#if 0
sprintf((char *)data, "%s,%c,%c,%c,%c,%c", data,
rpt_content.slot[num].ptp_en[i],
rpt_content.slot[num].ptp_delaytype[i],
rpt_content.slot[num].ptp_multicast[i],
rpt_content.slot[num].ptp_enp[i],
rpt_content.slot[num].ptp_step[i]);
#endif
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ptp_sync[i]);
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ptp_announce[i]);
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ptp_delayreq[i]);
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ptp_pdelayreq[i]);
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ptp_delaycom[i]);
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_linkmode[i]);
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_out[i]);
//2014.12.2
if(rpt_ptp_mtc.ptp_prio1[num][i][0] != 'A')
{
//printf("\nrpt_ptp_mtc.ptp_prio1[%d][%d][0] != A\n",num,i);
memset(rpt_ptp_mtc.ptp_prio1[num][i], 0, 5);
}
if(rpt_ptp_mtc.ptp_prio2[num][i][0] != 'B')
{
//printf("\nrpt_ptp_mtc.ptp_prio2[%d][%d][0] != B\n",num,i);
memset(rpt_ptp_mtc.ptp_prio2[num][i], 0, 5);
}
sprintf((char *)data, "%s,%s", data, rpt_ptp_mtc.ptp_prio1[num][i]);
sprintf((char *)data, "%s,%s", data, rpt_ptp_mtc.ptp_prio2[num][i]);
//sprintf((char *)data, "%s,%s", data, rpt_ptp_mtc.ptp_dom[num][i]);
#if 0
sprintf((char *)data, "%s,%s,%s,%s,%s,%s,%c,%c", data,
rpt_content.slot[num].ptp_sync[i],
rpt_content.slot[num].ptp_announce[i],
rpt_content.slot[num].ptp_delayreq[i],
rpt_content.slot[num].ptp_pdelayreq[i],
rpt_content.slot[num].ptp_delaycom[i],
rpt_content.slot[num].ptp_linkmode[i],
rpt_content.slot[num].ptp_out[i]);
#endif
}
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ntp_leap);
}
else if(slot_type[num] == 'j')
{
flag_1 = strlen(rpt_content.slot[num].sy_ver);
sprintf((char *)data, "%s%c,%c,%s", data, slot, slot_type[num],
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot[num].sy_ver));
for(i = 0; i < 4; i++)
{
sprintf((char *)data, "%s,%d,%s,%s,%s,%s", data, i + 1,
rpt_content.slot[num].ptp_mac[i],
rpt_content.slot[num].ptp_ip[i],
rpt_content.slot[num].ptp_mask[i],
rpt_content.slot[num].ptp_gate[i]
);
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_en[i]); //PTP Enable
sprintf((char *)data, "%s,%c", data, rpt_ptp_mtc.esmc_en[num][i]); //Esmc enable
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_delaytype[i]);//Delay type
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_multicast[i]);//multicast
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_enp[i]); //enp
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_step[i]); //step
//
//!
//
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ptp_sync[i]);
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ptp_announce[i]);
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ptp_delaycom[i]);
if(rpt_ptp_mtc.ptp_prio1[num][i][0] != 'A')
{
//printf("\nrpt_ptp_mtc.ptp_prio1[%d][%d][0] != A\n",num,i);
memset(rpt_ptp_mtc.ptp_prio1[num][i], 0, 5);
}
if(rpt_ptp_mtc.ptp_prio2[num][i][0] != 'B')
{
//printf("\nrpt_ptp_mtc.ptp_prio2[%d][%d][0] != B\n",num,i);
memset(rpt_ptp_mtc.ptp_prio2[num][i], 0, 5);
}
sprintf((char *)data, "%s,%s", data, rpt_ptp_mtc.ptp_prio1[num][i]);
sprintf((char *)data, "%s,%s", data, rpt_ptp_mtc.ptp_prio2[num][i]);
sprintf((char *)data, "%s,%s", data, rpt_ptp_mtc.ptp_dom[num][i]); //ptp domain
sprintf((char *)data, "%s,%s", data, rpt_ptp_mtc.ptp_ver[num][i]); //pt version
//
//!sfp modual .
//
sprintf((char *)data, "%s,%s,%s,%s,%s", data, rpt_ptp_mtc.ptp_mtc_ip[num][i],rpt_ptp_mtc.sfp_type[num][i],rpt_ptp_mtc.sfp_oplo[num][i],rpt_ptp_mtc.sfp_ophi[num][i]); //PTP mtc,sfp type,oplo,ophi
//
//!ptp status byte.
//
sprintf((char *)data, "%s,%s,%s,%s,%s", data, rpt_ptp_mtc.time_source[num][i],rpt_ptp_mtc.clock_class[num][i],rpt_ptp_mtc.time_acc[num][i],rpt_ptp_mtc.synce_ssm[num][i]);//time source,clock class,time acc,synce
}
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ntp_leap);
}
else if(slot_type[num] == 's')
{
flag_1 = strlen(rpt_content.slot[num].sy_ver);
sprintf((char *)data, "%s%c,%c,%s", data, slot, slot_type[num],
(flag_1 == 0) ? (unsigned char *)COMMA_3 : (rpt_content.slot[num].sy_ver));
sprintf((char *)data, "%s,%d,%s,%s,%s", data, 1, rpt_content.slot[num].ptp_ip[0],
rpt_content.slot[num].ptp_gate[0],
rpt_content.slot[num].ptp_mask[0]);
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_delaytype[0]);
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_multicast[0]);
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_enp[0]);
sprintf((char *)data, "%s,%c", data, rpt_content.slot[num].ptp_step[0]);
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ptp_delayreq[0]);
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ptp_pdelayreq[0]);
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ptp_clkcls[0]);
sprintf((char *)data, "%s,%s", data, rpt_content.slot[num].ntp_leap);
}
else if(slot_type[num] == 'T' || slot_type[num] == 'X')
{
flag_1 = strlen(rpt_content.slot[num].sy_ver);
sprintf((char *)data, "%s%c,%c,%s,%s,%s", data, slot, slot_type[num],
flag_1 == 0 ? (unsigned char *)(COMMA_3) : (rpt_content.slot[num].sy_ver),
rpt_content.slot[num].rs_en,
rpt_content.slot[num].rs_tzo);
}
else if(slot_type[num] == 'S')
{
/*<response message>::= "<aid>,<type>,[<version-MCU>],[<version-FPGA>], [<version-CPLD>],[<version-PCB>],
< priority1 >,< priority2>,<mode1>,<port1>,<mode2>,<port2>,
<tl1>,<tl2>,<tl3>,<tl4>,<tl5>,<tl6>,<tl7>,<tl8>,<ph1 >,
<ph2>,<ph3 >,<ph4>,<ph5 >,<ph6>,<ph7 >,<ph8>"*/
flag_1 = strlen(rpt_content.slot[num].sy_ver);
sprintf((char *)data, "%s%c,S,%s,", data, slot,
flag_1 == 0 ? (unsigned char *)(COMMA_3) : (rpt_content.slot[num].sy_ver));
flag_1 = strlen(rpt_content.slot[num].ref_mod1);
flag_2 = strlen(rpt_content.slot[num].ref_mod2);
sprintf((char *)data, "%s%s,%s,%s,%s,", data,
rpt_content.slot[num].ref_prio1,
rpt_content.slot[num].ref_prio2,
flag_1 == 0 ? (unsigned char *)(COMMA_1) : (rpt_content.slot[num].ref_mod1),
flag_2 == 0 ? (unsigned char *)(COMMA_1) : (rpt_content.slot[num].ref_mod2));
flag_1 = strlen(rpt_content.slot[num].ref_ph);
// flag_2=strlen(rpt_content.slot[num].ref_tl);
sprintf((char *)data, "%s%s,%s,%s,%s,%s,%s,%s,%c", data,
rpt_content.slot[num].ref_typ1,
rpt_content.slot[num].ref_typ2,
rpt_content.slot[num].ref_sa,
rpt_content.slot[num].ref_tl,
rpt_content.slot[num].ref_en,
flag_1 == 0 ? (unsigned char *)(COMMA_7) : (rpt_content.slot[num].ref_ph),
((0 == strlen(rpt_content.slot[num].ref_ssm_en)) ? (unsigned char *)("00000000") : rpt_content.slot[num].ref_ssm_en),rpt_content.slot[num].ref_2mb_lvlm);
// flag_2==0?(unsigned char *)(COMMA_7):(rpt_content.slot[num].ref_tl));
}
else if(slot_type[num] == 'U')
{
flag_1 = strlen(rpt_content.slot[num].sy_ver);
sprintf((char *)data, "%s%c,U,%s,%s", data, slot,
flag_1 == 0 ? (unsigned char *)(COMMA_3) : (rpt_content.slot[num].sy_ver),
rpt_content.slot[num].ppx_mod);
}
else if(slot_type[num] == 'V')
{
flag_1 = strlen(rpt_content.slot[num].sy_ver);
memset(tmp, 0, 4);
if((rpt_content.slot[num].tod_br < 0x30 ) || (rpt_content.slot[num].tod_br > 0x37))
{
sprintf((char *)tmp, ",%c", rpt_content.slot[num].tod_tzo);
}
else
{
sprintf((char *)tmp, "%c,%c", rpt_content.slot[num].tod_br, rpt_content.slot[num].tod_tzo);
}
sprintf((char *)data, "%s%c,V,%s,%s,%s", data, slot,
flag_1 == 0 ? (unsigned char *)(COMMA_3) : (rpt_content.slot[num].sy_ver),
rpt_content.slot[num].tod_en, tmp);
}
else if(slot_type[num] == 'W')
{
flag_1 = strlen(rpt_content.slot[num].sy_ver);
sprintf((char *)data, "%s%c,W,%s,%s,%s,%s", data, slot,
flag_1 == 0 ? (unsigned char *)(COMMA_3) : (rpt_content.slot[num].sy_ver),
rpt_content.slot[num].igb_en,
rpt_content.slot[num].igb_rat,
rpt_content.slot[num].igb_max);
}
else if(slot_type[num] == 'Z')
{
/*<ip>,<mac>,<gateway>,<netmask>,
<bcast_en>,<interval>,<tpv_en>,<md5_en>,<ois_en>,
[<keyid>,<md5key>]+*/
#if 1
flag_1 = strlen(rpt_content.slot[num].sy_ver);
sprintf((char *)data, "%s%c,Z,%s,", data, slot,
flag_1 == 0 ? (unsigned char *)(COMMA_3) : (rpt_content.slot[num].sy_ver));
if((rpt_content.slot[num].ntp_bcast_en == '1') || (rpt_content.slot[num].ntp_bcast_en == '0'))
{
sprintf((char *)tmp1, "%c,", rpt_content.slot[num].ntp_bcast_en);
tmp1[2] = '\0';
}
else
{
tmp1[0] = ',';
tmp1[1] = '\0';
}
if((rpt_content.slot[num].ntp_tpv_en >= '@') && (rpt_content.slot[num].ntp_tpv_en < 'H'))
{
sprintf((char *)tmp2, "%c,", rpt_content.slot[num].ntp_tpv_en);
tmp2[2] = '\0';
}
else
{
tmp2[0] = ',';
tmp2[1] = '\0';
}
if((rpt_content.slot[num].ntp_md5_en == '1') || (rpt_content.slot[num].ntp_md5_en == '0'))
{
sprintf((char *)tmp3, "%c,", rpt_content.slot[num].ntp_md5_en);
tmp3[2] = '\0';
}
else
{
tmp3[0] = ',';
tmp3[1] = '\0';
}
if((rpt_content.slot[num].ntp_leap[0] >= '0') && (rpt_content.slot[num].ntp_leap[0] <= '9'))
{
strncpy((char *)tmp4,rpt_content.slot[num].ntp_leap,2);
tmp4[2] = '\0';
}
else
{
tmp4[0] = '\0';
}
sprintf((char *)data, "%s%s%s,%s%s%s", data,
tmp1,
rpt_content.slot[num].ntp_interval,
tmp2,
tmp3,
tmp4);
if(rpt_content.slot[num].ntp_mcu[0] < '0' || rpt_content.slot[num].ntp_mcu[0] >'9')
{
memset(rpt_content.slot[num].ntp_mcu,0,sizeof(rpt_content.slot[num].ntp_mcu));
}
__srtcat(data,rpt_content.slot[num].ntp_mcu);
__srtcat(data,rpt_content.slot[num].ntp_portEn);
__srtcat(data,rpt_content.slot[num].ntp_portStatus);
for(i = 0; i < 12; i++)
{
tmp1[0] = i+'1';
tmp1[1] = 0;
if(9 == i)
{
tmp1[0] = '1';
tmp1[1] = '0';
}
else if(10 == i)
{
tmp1[0] = '1';
tmp1[1] = '1';
}
if(11 == i)
{
tmp1[0] = '1';
tmp1[1] = '2';
}
__srtcat(data,tmp1);
strcat_mac(data,num,i);
strcat_ip(data,num,i);
strcat_gate(data,num,i);
strcat_mask(data,num,i);
}
for(i = 0; i < 4; i++)
{
length = strlen(data);
if(0 == i)
sprintf(&data[length],",%s,%s,",rpt_content.slot[num].ntp_keyid[i],rpt_content.slot[num].ntp_key[i]);
else if(3 == i)
sprintf(&data[length],"%s,%s",rpt_content.slot[num].ntp_keyid[i],rpt_content.slot[num].ntp_key[i]);
else
sprintf(&data[length],"%s,%s,",rpt_content.slot[num].ntp_keyid[i],rpt_content.slot[num].ntp_key[i]);
}
#else
sprintf(data,"%s%s",data,"i,Z,V01.04,V02.01,,V00.01,1,0064,D,0,17,9,OO@O,OO@O,1,AABBCCDDEEFF,L@JH@OA@,L@JH@O@A,OOOOOO@@,2,AABBCCDDEEFF,L@JH@OA@,L@JH@O@A,OOOOOO@@,3,AABBCCDDEEFF,L@JH@OA@,L@JH@O@A,OOOOOO@@,4,AABBCCDDEEFF,L@JH@OA@,L@JH@O@A,OOOOOO@@,5,AABBCCDDEEFF,L@JH@OA@,L@JH@O@A,OOOOOO@@,6,AABBCCDDEEFF,L@JH@OA@,L@JH@O@A,OOOOOO@@,7,AABBCCDDEEFF,L@JH@OA@,L@JH@O@A,OOOOOO@@,8,AABBCCDDEEFF,L@JH@OA@,L@JH@O@A,OOOOOO@@,9,AABBCCDDEEFF,L@JH@OA@,L@JH@O@A,OOOOOO@@,10,AABBCCDDEEFF,L@JH@OA@,L@JH@O@A,OOOOOO@@,11,AABBCCDDEEFF,L@JH@OA@,L@JH@O@A,OOOOOO@@,12,AABBCCDDEEFF,L@JH@OA@,L@JH@O@A,OOOOOO@@");
//sprintf(data,"%s%s",data,"j,Z,V01.04,V02.01,,,1,0064,D,0,17,9,,,1,L@JH@OA@,AABBCCDDEEFF,L@JH@O@A,OOOOOO@@");
#endif
}
else if('m' == slot_type[num])
{
sprintf((char *)data, "%s%c,m", data, slot);
get_sta1_ver(data, num);
//sprintf((char *)data,"%s%c,m,%s,%s,,1,JLABAE@C,JLABAE@A,OOOOOO@@,,,",data,slot,"V01.00","V01.00");
}
}
void rpt_test(char slot, unsigned char *data)
{
int num = (int)(slot - 'a');
if((num >= 0) && (num < 14))
{
sprintf((char *)data, "%s%c,Y,%s", data, slot, rpt_content.slot[num].sy_ver);
}
else if(num == 14)
{
sprintf((char *)data, "%s%c,Y,%s", data, slot, rpt_content.slot_o.sy_ver);
}
else if(num == 15)
{
sprintf((char *)data, "%s%c,Y,%s", data, slot, rpt_content.slot_p.sy_ver);
}
else if(num == 16)
{
sprintf((char *)data, "%s%c,Y,%s", data, slot, rpt_content.slot_q.sy_ver);
}
else if(num == 17)
{
sprintf((char *)data, "%s%c,Y,%s", data, slot, rpt_content.slot_r.sy_ver);
}
}
void rpt_drv(char slot, unsigned char *data)
{
int num = (int)(slot - 'a');
if( (12 == num) || (13 == num) )
{
sprintf((char *)data, "%s%c,d,%s,,,%d,%d,%d",
data, slot,
((0 == strlen(gExtCtx.drv[num - 12].drvVer)) ? (char *)"," : (char *)(gExtCtx.drv[num - 12].drvVer)),
gExtCtx.drv[num - 12].drvPR[0],
gExtCtx.drv[num - 12].drvPR[1],
gExtCtx.drv[num - 12].drvPR[2]);
}
}
void rpt_mcp(unsigned char *data)
{
/*“<aid>,<type>,[<version-MCU>],[<version-FPGA>], [<version-CPLD>],[<version-PCB>],
<ip>,<gateway>,<netmask>, < dns1>,< dns2>,<mac>,<source>,<leapnum>”*/
unsigned char mcp_ssm_th = 0;
unsigned char mcp_ip[16];
unsigned char mcp_gateway[16];
unsigned char mcp_mask[16];
unsigned char mcp_mac[18];
//int len;
memset( mcp_ip, '\0', sizeof(unsigned char) * 16);
memset( mcp_gateway, '\0', sizeof(unsigned char) * 16);
memset( mcp_mask, '\0', sizeof(unsigned char) * 16);
memset( mcp_mac, '\0', sizeof(unsigned char) * 18);
API_Get_McpIp(mcp_ip);
API_Get_McpGateWay(mcp_gateway);
//printf("gateway=%s\n",mcp_gateway);
if(if_a_string_is_a_valid_ipv4_address(mcp_gateway) == 0)
{}
else
{
memset( mcp_gateway, '\0', sizeof(unsigned char) * 16);
}
API_Get_McpMask(mcp_mask);
API_Get_McpMacAddr(mcp_mac);
if(if_a_string_is_a_valid_ipv4_address(conf_content.slot_u.dns1) == 0)
{}
else
{
memset( conf_content.slot_u.dns1, '\0', sizeof(unsigned char) * 16);
}
if(if_a_string_is_a_valid_ipv4_address(conf_content.slot_u.dns2) == 0)
{}
else
{
memset( conf_content.slot_u.dns2, '\0', sizeof(unsigned char) * 16);
}
sprintf((char *)data, "%su,N,%s,%s,,,%s,%s,%s,%s,%s,%s", data, (unsigned char *)MCP_VER,
fpga_ver,
mcp_ip, mcp_gateway, mcp_mask,
conf_content.slot_u.dns1,
conf_content.slot_u.dns2, mcp_mac);
switch(conf_content.slot_u.out_ssm_oth)
{
case 0x02:
mcp_ssm_th = 'a';
break;
case 0x04:
mcp_ssm_th = 'b';
break;
case 0x08:
mcp_ssm_th = 'c';
break;
case 0x0b:
mcp_ssm_th = 'd';
break;
case 0x0f:
mcp_ssm_th = 'e';
break;
case 0x00:
mcp_ssm_th = 'f';
break;
}
#if 0
sprintf((char *)data, "%s,%c,%s,%c,%s,%c,%c", data,
conf_content.slot_u.time_source,
conf_content.slot_u.leap_num,
conf_content.slot_u.fb,
conf_content.slot_u.tl,
conf_content.slot_u.out_ssm_en,
mcp_ssm_th);
#endif
//2014-6-19
sprintf((char *)data, "%s,%c", data, conf_content.slot_u.time_source);
sprintf((char *)data, "%s,%s", data, conf_content.slot_u.leap_num);
sprintf((char *)data, "%s,%c", data, conf_content.slot_u.fb);
sprintf((char *)data, "%s,%s", data, conf_content.slot_u.tl);
sprintf((char *)data, "%s,%c", data, conf_content.slot_u.out_ssm_en);
sprintf((char *)data, "%s,%c,%c", data, mcp_ssm_th,conf_content3.mcp_protocol);
sprintf((char *)data, "%s,%s%c%s", data, mcp_date,SP,mcp_time);
//2014.12.9
/*
if(conf_content3.LeapMod !='0' && conf_content3.LeapMod != '1')
{
conf_content3.LeapMod = '0';
save_config();
}
sprintf((char *)data,"%s,%c",data,conf_content3.LeapMod);
*/
}
void get_gbtp_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 0x00:
case 0x01:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
case 0x02:
case 0x03:
case 0x04:
case 0x05:
case 0x06:
case 0x07:
break;
}
}
void get_btp_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 0x01:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
case 0x00:
case 0x02:
case 0x03:
case 0x04:
case 0x05:
case 0x06:
case 0x07:
break;
}
}
void get_gtp_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 0x00:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
case 0x01:
case 0x02:
case 0x03:
case 0x04:
case 0x05:
case 0x06:
case 0x07:
break;
}
}
void get_rb_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 0:
case 1:
case 2:
case 3:
case 4:
case 5:
case 6:
case 7:
case 8:
case 9:
case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
case 16:
case 17:
case 18:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
default:
break;
}
}
void get_TP16_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 00:
case 01:
case 02:
case 03:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
/*case 04:
case 05:
case 06:
case 07:
case 8:
case 9:
case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
case 16:
case 17:
case 18:
case 19:
case 20:
{
memcpy(ntfcncde, TYPE_MJ, 2);
memcpy(almcde, TYPE_MJ_A, 2);
break;
}
case 21:
case 22:
case 23:
break;*/
default:
break;
}
}
void get_OUT16_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
/*case 00:
case 01:
case 18:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
case 02:
case 03:
case 04:
case 05:
case 06:
case 07:
case 8:
case 9:
case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
case 16:
case 17:
{
memcpy(ntfcncde, TYPE_MJ, 2);
memcpy(almcde, TYPE_MJ_A, 2);
break;
}
case 19:
case 20:
case 21:
case 22:
case 23:
break;*/
default:
break;
}
}
void get_PTP_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
/*case 00:
case 01:
case 02:
case 03:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
case 04:
case 05:
case 06:
case 07:
case 8:
case 9:
case 10:
case 11:
case 12:
{
memcpy(ntfcncde, TYPE_MJ, 2);
memcpy(almcde, TYPE_MJ_A, 2);
break;
}
case 13:
case 14:
case 15:
break;*/
default:
break;
}
}
/***************************FUNCTION :get_PGEIN_alm_type********************************/
/***************************PARAMETERS: ********************************/
void get_PGEIN_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 00:
{
memcpy(ntfcncde, TYPE_MJ, 2);
memcpy(almcde, TYPE_MJ_A, 2);
break;
}
/*case 01:
case 02:
case 03:
case 04:
case 05:
case 06:
case 07:
case 8:
case 9:
case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
break;*/
default:
break;
}
}
void get_PGE4V_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 00:
case 01:
case 02:
case 03:
case 04:
case 05:
case 06:
case 07:
case 8:
case 9:
// case 08:
// case 09:
case 16:
case 17:
case 24:
case 25:
case 32:
case 33:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
/*case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
break;*/
default:
break;
}
}
void get_GBTPIIV2_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 00:
case 02:
case 03:
case 04:
{
memcpy(ntfcncde, TYPE_MJ, 2);
memcpy(almcde, TYPE_MJ_A, 2);
break;
}
case 01:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
/*case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
break;*/
default:
break;
}
}
void get_drv_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 00:
case 01:
case 02:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
/*
case 03:
case 04:
case 05:
case 06:
case 07:
case 08:
case 09:
case 16:
case 17:
case 24:
case 25:
case 32:
case 33:
case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
break;*/
default:
break;
}
}
void get_mge_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 00:
case 01:
case 02:
case 03:
case 04:
case 05:
case 06:
case 07:
case 8:
case 9:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
case 10:
case 11:
{
memcpy(ntfcncde, TYPE_MJ, 2);
memcpy(almcde, TYPE_MJ_A, 2);
break;
}
/*
case 03:
case 04:
case 05:
case 06:
case 07:
case 08:
case 09:
case 16:
case 17:
case 24:
case 25:
case 32:
case 33:
case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
break;*/
default:
break;
}
}
void get_tod_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 00:
case 01:
case 02:
case 03:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
/*case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
break;*/
default:
break;
}
}
void get_ntp_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 00:
case 01:
case 02:
case 03:
case 04:
case 05:
case 06:
case 07:
case 8:
case 9:
case 10:
case 11:
case 12:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
/*case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
break;*/
default:
break;
}
}
void get_MCP_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 0:
case 1:
case 4:
case 5:
{
memcpy(ntfcncde, TYPE_MJ, 2);
memcpy(almcde, TYPE_MJ_A, 2);
break;
}
case 6:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
/*case 2:
case 3:
case 6:
case 7:
case 8:
case 9:
case 10:
case 11:
case 12:
case 13:
case 14:
case 15:
case 16:
case 17:
case 18:
case 19:
case 20:
case 21:
case 22:
case 23:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}*/
default:
break;
}
}
void get_REF_alm_type(unsigned char *ntfcncde, unsigned char *almcde, int num)
{
switch(num)
{
case 00:
case 01:
case 02:
case 03:
case 12:
case 04:
case 05:
case 06:
case 07:
case 8:
case 9:
case 10:
case 11:
case 13:
case 14:
case 15:
case 16:
case 17:
case 18:
case 19:
case 20:
case 21:
case 22:
case 23:
case 24:
case 25:
case 26:
case 27:
case 28:
case 29:
case 30:
case 31:
case 32:
case 33:
case 34:
case 35:
case 36:
case 37:
case 38:
case 39:
case 40:
case 41:
case 42:
case 43:
case 44:
case 45:
case 46:
case 47:
{
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
break;
}
default:
break;
}
}
int get_format(char *format, unsigned char *ctag)
{
unsigned char tmp[MAXDATASIZE];
int len = 0;
memset(tmp, '\0', MAXDATASIZE);
get_time_fromnet();
sprintf((char *)tmp, "%c%c%c%c%c%c%s%c", CR, LF, LF, SP, SP, SP, conf_content.slot_u.tid, SP);
sprintf((char *)tmp, "%s%s%c%s", tmp, mcp_date, SP, mcp_time);
sprintf((char *)tmp, "%s%c%cM%c%c%s%cCOMPLD%c%c", tmp, CR, LF, SP, SP, ctag, SP, CR, LF);
len = strlen(tmp);
memcpy(format, tmp, len);
return len;
}
void get_alm(char slot, unsigned char *data)
{
int num, i;
num = (int)(slot - 'a');
//printf("<get_alm>:%d\n",num);
if( (num >= 0) && (num < 23) )
{
if( (num == 18) || (num == 19) ) //电源
{
if(slot_type[num] != 'O')
{
sprintf((char *)data, "%s%c%c%c\"%c,M,\"%c%c", data, SP, SP, SP, slot, CR, LF);
}
}
else if(num == 21)
{
}
else
{
if(slot_type[num] != 'O')
{
sprintf((char *)data, "%s%c%c%c%s%c%c", data, SP, SP, SP, alm_sta[num], CR, LF);
}
//
//!reftf alarm : @@@@@@ PPPPPP
//
if((slot_type[num] == 'S') && (num == 0))
{
sprintf((char *)data, "%s%c%c%c%s%c%c;", data, SP, SP, SP, alm_sta[18], CR, LF);
}
else if((slot_type[num] == 'S') && (num == 1))
{
sprintf((char *)data, "%s%c%c%c%s%c%c;", data, SP, SP, SP, alm_sta[19], CR, LF);
}
//
//!pge4v2 alarm : @@@@@@ PPPPPP
//
else if(slot_type[num] == 'j')
{
sprintf((char *)data, "%s%c%c%c%s%c%c;", data, SP, SP, SP, alm_sta[PGE4S_ALM5_START+num], CR, LF);
}
else
{
sprintf((char *)data, "%s;", data);
}
}
}
else if( (slot == ':') || (slot == ' ') )
{
for(i = 0; i < 18; i++)
{
if(slot_type[i] != 'O')
{
if( (slot_type[i] == 'd' ) && (12 == i || 13 == i) )
{
//20140926改变drv在线告警
alm_sta[i][5] &= gExtCtx.save.onlineSta;
}
else
{
;
}
if(alm_sta[i][0] != '\0')
sprintf((char *)data, "%s%c%c%c%s%c%c", data, SP, SP, SP, alm_sta[i], CR, LF);
}
//
//! reftf @@@@@@ PPPPPP
//
if((slot_type[i] == 'S') && (i == 0))
{
sprintf((char *)data, "%s%c%c%c%s%c%c", data, SP, SP, SP, alm_sta[18], CR, LF);
}
else if((slot_type[i] == 'S') && (i == 1))
{
sprintf((char *)data, "%s%c%c%c%s%c%c", data, SP, SP, SP, alm_sta[19], CR, LF);
}
//
//! pge4v2 @@@@@@ PPPPPP
//
else if(slot_type[i] == 'j')
{
sprintf((char *)data, "%s%c%c%c%s%c%c", data, SP, SP, SP, alm_sta[PGE4S_ALM5_START+i], CR, LF);
}
}
if(slot_type[22] != 'O')
{
sprintf((char *)data, "%s%c%c%c%s%c%c", data, SP, SP, SP, alm_sta[20], CR, LF);
sprintf((char *)data, "%s%c%c%c%s%c%c;", data, SP, SP, SP, alm_sta[22], CR, LF);
}
else
{
sprintf((char *)data, "%s%c%c%c%s%c%c;", data, SP, SP, SP, alm_sta[20], CR, LF);
}
}
}
void get_ext_alm(char slot, unsigned char *data)
{
int i;
int opt = 0;
if( (slot == ':') || (slot == ' ') )
{
for(i = 0; i < 10; i++)
{
if( (EXT_NONE != gExtCtx.extBid[0][i]) &&
(0 != memcmp(gExtCtx.out[i].outAlm, "@@@@@", 5)))
{
opt = 1;
sprintf((char *)data,
"%s%c%c%c\"%c,%c,%s\"\r\n",
data,
SP, SP, SP,
i + 'a',
gExtCtx.extBid[0][i],
gExtCtx.out[i].outAlm);
}
}
if( (EXT_NONE != gExtCtx.extBid[0][10]) &&
(0 != memcmp(gExtCtx.mgr[0].mgrAlm, "@@@@@@@", 7)))
{
opt = 1;
sprintf((char *)data,
"%s%c%c%c\"%c,%c,%s\"\r\n",
data,
SP, SP, SP,
'k',
gExtCtx.extBid[0][10],
gExtCtx.mgr[0].mgrAlm);
}
if( (EXT_NONE != gExtCtx.extBid[0][11]) &&
(0 != memcmp(gExtCtx.mgr[1].mgrAlm, "@@@@@@@", 7)))
{
opt = 1;
sprintf((char *)data,
"%s%c%c%c\"%c,%c,%s\"\r\n",
data,
SP, SP, SP,
'l',
gExtCtx.extBid[0][11],
gExtCtx.mgr[1].mgrAlm);
}
if(0 == opt)
{
strcat(data, " \"\"\r\n");
}
strcat(data, "/\r\n");
opt = 0;
for(i = 0; i < 10; i++)
{
if( (EXT_NONE != gExtCtx.extBid[1][i]) &&
(0 != memcmp(gExtCtx.out[10 + i].outAlm, "@@@@@", 5)))
{
opt = 1;
sprintf((char *)data,
"%s%c%c%c\"%c,%c,%s\"\r\n",
data, SP, SP, SP,
i + 'a',
gExtCtx.extBid[1][i],
gExtCtx.out[10 + i].outAlm);
}
}
if( (EXT_NONE != gExtCtx.extBid[1][10]) &&
(0 != memcmp(gExtCtx.mgr[2].mgrAlm, "@@@@@@@", 7)))
{
opt = 1;
sprintf((char *)data,
"%s%c%c%c\"%c,%c,%s\"\r\n",
data, SP, SP, SP, 'k',
gExtCtx.extBid[1][10],
gExtCtx.mgr[2].mgrAlm);
}
if( (EXT_NONE != gExtCtx.extBid[1][11]) &&
(0 != memcmp(gExtCtx.mgr[3].mgrAlm, "@@@@@@@", 7)))
{
opt = 1;
sprintf((char *)data,
"%s%c%c%c\"%c,%c,%s\"\r\n",
data, SP, SP, SP, 'l',
gExtCtx.extBid[1][11],
gExtCtx.mgr[3].mgrAlm);
}
if(0 == opt)
{
strcat(data, " \"\"\r\n");
}
strcat(data, "/\r\n");
opt = 0;
for(i = 0; i < 10; i++)
{
if( (EXT_NONE != gExtCtx.extBid[2][i]) &&
(0 != memcmp(gExtCtx.out[20 + i].outAlm, "@@@@@", 5)))
{
opt = 1;
sprintf((char *)data,
"%s%c%c%c\"%c,%c,%s\"\r\n",
data, SP, SP, SP, i + 'a',
gExtCtx.extBid[2][i],
gExtCtx.out[20 + i].outAlm);
}
}
if( (EXT_NONE != gExtCtx.extBid[2][10]) &&
(0 != memcmp(gExtCtx.mgr[4].mgrAlm, "@@@@@@@", 7)))
{
opt = 1;
sprintf((char *)data,
"%s%c%c%c\"%c,%c,%s\"\r\n",
data, SP, SP, SP, 'k',
gExtCtx.extBid[2][10],
gExtCtx.mgr[4].mgrAlm);
}
if( (EXT_NONE != gExtCtx.extBid[2][11]) &&
(0 != memcmp(gExtCtx.mgr[5].mgrAlm, "@@@@@@@", 7)))
{
opt = 1;
sprintf((char *)data,
"%s%c%c%c\"%c,%c,%s\"\r\n",
data, SP, SP, SP, 'l',
gExtCtx.extBid[2][11],
gExtCtx.mgr[5].mgrAlm);
}
if(0 == opt)
{
strcat(data, " \"\"\r\n");
}
strcat(data, ";");
}
}
void get_ext1_alm(char slot, unsigned char *data)
{
int num, i;
if( (slot == ':') || (slot == ' ') )
{
for(i = 0; i < 10; i++)
{
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, i + 'a', gExtCtx.extBid[0][i]);
if(EXT_NONE != gExtCtx.extBid[0][i])
{
sprintf((char *)data, "%s,%s\"%c%c", data, gExtCtx.out[i].outAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n");
}
}
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, 'k', gExtCtx.extBid[0][10]);
if(EXT_NONE != gExtCtx.extBid[0][10])
{
sprintf((char *)data, "%s,%s\"%c%c", data, gExtCtx.mgr[0].mgrAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n");
}
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, 'l', gExtCtx.extBid[0][11]);
if(EXT_NONE != gExtCtx.extBid[0][11])
{
sprintf((char *)data, "%s,%s\"%c%c;", data, gExtCtx.mgr[1].mgrAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n;");
}
}
else
{
num = (int)(slot - 'a');
if( (num >= 0) && (num <= 9) )
{
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, slot, gExtCtx.extBid[0][num]);
if(EXT_NONE != gExtCtx.extBid[0][num])
{
sprintf((char *)data, "%s,%s\"%c%c;", data, gExtCtx.out[num].outAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n;");
}
}
else if(10 == num)
{
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, slot, gExtCtx.extBid[0][num]);
if(EXT_NONE != gExtCtx.extBid[0][num])
{
sprintf((char *)data, "%s,%s\"%c%c;", data, gExtCtx.mgr[0].mgrAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n;");
}
}
else if(11 == num)
{
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, slot, gExtCtx.extBid[0][num]);
if(EXT_NONE != gExtCtx.extBid[0][num])
{
sprintf((char *)data, "%s,%s\"%c%c;", data, gExtCtx.mgr[1].mgrAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n;");
}
}
else
{
//
}
}
}
void get_ext2_alm(char slot, unsigned char *data)
{
int num, i;
if( (slot == ':') || (slot == ' ') )
{
for(i = 0; i < 10; i++)
{
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, i + 'a', gExtCtx.extBid[1][i]);
if(EXT_NONE != gExtCtx.extBid[1][i])
{
sprintf((char *)data, "%s,%s\"%c%c", data, gExtCtx.out[10 + i].outAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n");
}
}
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, 'k', gExtCtx.extBid[1][10]);
if(EXT_NONE != gExtCtx.extBid[1][10])
{
sprintf((char *)data, "%s,%s\"%c%c", data, gExtCtx.mgr[2].mgrAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n");
}
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, 'l', gExtCtx.extBid[1][11]);
if(EXT_NONE != gExtCtx.extBid[1][11])
{
sprintf((char *)data, "%s,%s\"%c%c;", data, gExtCtx.mgr[3].mgrAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n;");
}
}
else
{
num = (int)(slot - 'a');
if( (num >= 0) && (num <= 9) )
{
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, slot, gExtCtx.extBid[1][num]);
if(EXT_NONE != gExtCtx.extBid[1][num])
{
sprintf((char *)data, "%s,%s\"%c%c;", data, gExtCtx.out[10 + num].outAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n;");
}
}
else if(10 == num)
{
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, slot, gExtCtx.extBid[1][num]);
if(EXT_NONE != gExtCtx.extBid[1][num])
{
sprintf((char *)data, "%s,%s\"%c%c;", data, gExtCtx.mgr[2].mgrAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n;");
}
}
else if(11 == num)
{
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, slot, gExtCtx.extBid[1][num]);
if(EXT_NONE != gExtCtx.extBid[1][num])
{
sprintf((char *)data, "%s,%s\"%c%c;", data, gExtCtx.mgr[3].mgrAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n;");
}
}
else
{
//
}
}
}
void get_ext3_alm(char slot, unsigned char *data)
{
int num, i;
if( (slot == ':') || (slot == ' ') )
{
for(i = 0; i < 10; i++)
{
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, i + 'a', gExtCtx.extBid[2][i]);
if(EXT_NONE != gExtCtx.extBid[2][i])
{
sprintf((char *)data, "%s,%s\"%c%c", data, gExtCtx.out[20 + i].outAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n");
}
}
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, 'k', gExtCtx.extBid[2][10]);
if(EXT_NONE != gExtCtx.extBid[2][10])
{
sprintf((char *)data, "%s,%s\"%c%c", data, gExtCtx.mgr[4].mgrAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n");
}
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, 'l', gExtCtx.extBid[2][11]);
if(EXT_NONE != gExtCtx.extBid[2][11])
{
sprintf((char *)data, "%s,%s\"%c%c;", data, gExtCtx.mgr[5].mgrAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n;");
}
}
else
{
num = (int)(slot - 'a');
if( (num >= 0) && (num <= 9) )
{
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, slot, gExtCtx.extBid[2][num]);
if(EXT_NONE != gExtCtx.extBid[2][num])
{
sprintf((char *)data, "%s,%s\"%c%c;", data, gExtCtx.out[20 + num].outAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n;");
}
}
else if(10 == num)
{
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, slot, gExtCtx.extBid[2][num]);
if(EXT_NONE != gExtCtx.extBid[2][num])
{
sprintf((char *)data, "%s,%s\"%c%c;", data, gExtCtx.mgr[4].mgrAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n;");
}
}
else if(11 == num)
{
sprintf((char *)data, "%s%c%c%c\"%c,%c", data, SP, SP, SP, slot, gExtCtx.extBid[2][num]);
if(EXT_NONE != gExtCtx.extBid[2][num])
{
sprintf((char *)data, "%s,%s\"%c%c;", data, gExtCtx.mgr[5].mgrAlm, CR, LF);
}
else
{
strcat(data, "\"\r\n;");
}
}
else
{
//
}
}
}
/*********************Function:save_one_alm********************/
/*********************Parmeters: slot *******************/
/*********************Modify:2017/7/19 *******************/
void save_alm(char slot, unsigned char *data, int flag)
{
int num;
num = (int)(slot - 'a');
if(flag == 1) //REF
{
memcpy(alm_sta[num + 18], data, strlen(data));
}
else if(flag == 2)
{
memcpy(alm_sta[num + PGE4S_ALM5_START], data, strlen(data));
}
else if((num >= 0) && (num < 18))
{
/*if(0 == num || 1 == num)
{
printf("<ref alm %d>:'0x4':%s\n", num, data);
}*/
memcpy(alm_sta[num], data, strlen(data));
}
else if(num == 20)
{
memcpy(alm_sta[20], data, strlen(data));
}
else if(num == 22)
{
memcpy(alm_sta[22], data, strlen(data));
}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:<%d>:%s;\n", NET_INFO, num + 1, data);
}
#endif
}
int send_pullout_alm(int num, int flag_cl)
{
unsigned char alm[3];
unsigned char tmp[50];
unsigned char ntfcncde[3];
unsigned char almcde[3];
alm[0] = ((num & 0x0f) | 0x50); // 0x6x di 4 wei
if(flag_cl == 0)
{
alm[1] = (((num & 0x70) >> 3) | 0x50);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_MN, 2);
memcpy(almcde, TYPE_MN_A, 2);
rpt_alm_framing('u', TYPE_MN, TYPE_MN_A, alm);
}
else
{
alm[1] = (((num & 0x70) >> 3) | 0x51);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_CL, 2);
memcpy(almcde, TYPE_CL_A, 2);
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
get_time_fromnet();
sprintf((char *)tmp, "\"u:N,%s,%s,%s,%s,%s,NSA\"%c%c%c%c%c",
mcp_date, mcp_time, almcde, ntfcncde, alm, CR, LF, SP, SP, SP);
strncpy(saved_alms[saved_alms_cnt], tmp, strlen(tmp));
saved_alms_cnt++;
if(saved_alms_cnt == SAVE_ALM_NUM)
{
saved_alms_cnt = 0;
}
/******/
return 0;
}
void send_alm(char slot, int num, char type, int flag_cl)
{
unsigned char ntfcncde[3];
unsigned char almcde[3];
unsigned char alm[3];
unsigned char tmp[50];
//unsigned char act_almbit = 0;
memset(ntfcncde, '\0', 3);
memset(almcde, '\0', 3);
memset(alm, '\0', 3);
memset(tmp, '\0', 50);
if(type == 'L' ||'e' == type || 'g' == type )
{
get_gbtp_alm_type(ntfcncde, almcde, num);
}
else if( 'l' == type)
{
get_btp_alm_type(ntfcncde, almcde, num);
}
else if( type == 'P')
{
get_gtp_alm_type(ntfcncde, almcde, num);
}
//else if(type=='P')
// {
// get_gbtp_alm_type(ntfcncde,almcde,num);
//}
else if(type == 'K' || type == 'R')
{
get_rb_alm_type(ntfcncde, almcde, num);
}
else if(type == 'J' || type == 'a' || type == 'h' || type == 'i')
{
get_OUT16_alm_type(ntfcncde, almcde, num);
}
else if(type == 'I')
{
get_TP16_alm_type(ntfcncde, almcde, num);
}
else if(type == 'S')
{
get_REF_alm_type(ntfcncde, almcde, num);
}
else if((type < 'I') && (type > '@'))
{
get_PTP_alm_type(ntfcncde, almcde, num);
}
else if(type == 'Q')
{
memcpy(ntfcncde, TYPE_MJ, 2);
memcpy(almcde, TYPE_MJ_A, 2);
}
else if(type == 'N')
{
get_MCP_alm_type(ntfcncde, almcde, num);
}
else if(type == 'V')
{
get_tod_alm_type(ntfcncde, almcde, num);
}
else if(type == 'Z')
{
get_ntp_alm_type(ntfcncde, almcde, num);
}
else if(type == 's')
{
get_PGEIN_alm_type(ntfcncde, almcde, num);
}
else if(type == 'j')
{
get_PGE4V_alm_type(ntfcncde, almcde, num);
}
else if(type == 'd')
{
get_drv_alm_type(ntfcncde, almcde, num);
}
else if(type == 'f')
{
get_mge_alm_type(ntfcncde, almcde, num);
}
else if(type == 'v')
{
get_GBTPIIV2_alm_type(ntfcncde, almcde, num);
}
//num=num+1; 0110 Z3Z2Z1Z0 0110 Z7 Z6Z5Z4
//@@@@@@@@@@@@@@@@@@
alm[0] = ((num & 0x0f) | 0x50);
if(flag_cl == 0)
{
alm[1] = (((num & 0x70) >> 3) | 0x50);
memcpy(ntfcncde, TYPE_CL, 2);
memcpy(almcde, TYPE_CL_A, 2);
}
else
{
alm[1] = (((num & 0x70) >> 3) | 0x51);
}
alm[2] = '\0';
/******/
get_time_fromnet();
sprintf((char *)tmp, "\"%c:%c,%s,%s,%s,%s,%s,NSA\"%c%c%c%c%c",
slot, type, mcp_date, mcp_time, almcde, ntfcncde, alm, CR, LF, SP, SP, SP);
strncpy(saved_alms[saved_alms_cnt], tmp, strlen(tmp));
saved_alms_cnt++;
if(saved_alms_cnt == SAVE_ALM_NUM)
{
saved_alms_cnt = 0;
}
/******/
if(ntfcncde[0] != '\0')
rpt_alm_framing(slot, ntfcncde, almcde, alm);
}
void rpt_alm_to_client(char slot, unsigned char *data)
{
int num, i, j, len;
char tmp, type_flag, temp;
int star_ind = 0;
char errflag = 0;
len = strlen(data);
num = (int)(slot - 'a');
type_flag = slot_type[num];
//
//屏蔽掉多余告警
//
almMsk_cmdRALM(num, data, len);
if(num == 20)
{
num = 18; //mcp
}
else if(num == 22)
{
num = 19; //fan
}
//
//!0x04 第一行报警,0x05 第二行报警, 每行24报警
//
for(i = 0; i < len; i++)
{
if((data[0] >> 4) == 0x04)
{
tmp = (data[i] & 0x0f);
for(j = 0; j < 4; j++)
{
temp = ((tmp >> j) & 0x01);
if((temp == 0x00) && (flag_alm[num][i * 4 + j] == 0x01))
{
/*清除告警*/
send_alm(slot, (i * 4 + j), type_flag, 0);
}
else if((temp == 0x01) && (flag_alm[num][i * 4 + j] == 0x00))
{
/*上报告警*/
send_alm(slot, (i * 4 + j), type_flag, 1);
}
flag_alm[num][i * 4 + j] = temp;
}
}
else if((data[0] >> 4) == 0x05) //ref
{
//printf("<rpt_alm_to_client>:'ref num':%d\n",num);
//! flag_alm 20 ref1 21 ref2 (0x50)
switch(type_flag)
{
case 'S':
{
if((num != 1) || (num != 0))
errflag = 1;
else
star_ind = (int)PGE4S_TALM5_START + num - 2;
}
break;
case 'j':
{
if((num < 0) || (num > 13))
errflag = 1;
else
star_ind = (int)PGE4S_TALM5_START + num;
}
break;
default:
errflag = 1;
break;
}
if(errflag == 1)
return ;
tmp = (data[i] & 0x0f);
for(j = 0; j < 4; j++)
{
temp = ((tmp >> j) & 0x01);
if((temp == 0x00) && (flag_alm[star_ind][i * 4 + j] == 0x01))
{
/*清除告警*/
send_alm(slot, (i * 4 + j + 24), type_flag, 0);
}
else if((temp == 0x01) && (flag_alm[star_ind][i * 4 + j] == 0x00))
{
/*上报告警*/
send_alm(slot, (i * 4 + j + 24), type_flag, 1);
}
flag_alm[star_ind][i * 4 + j] = temp;
}
}
}
}
void respond_success(int client, unsigned char *ctag)
{
int len, send_ok;
unsigned char sendbuf[MAXDATASIZE];
memset(sendbuf, '\0', MAXDATASIZE);
len = get_format(sendbuf, ctag);
sprintf((char *)sendbuf, "%s;", sendbuf);
send_ok = send(client, sendbuf, len + 1, MSG_NOSIGNAL);
if(send_ok == -1)
{
/* close(client);
FD_CLR(client, &allset);
client= -1;*/
printf("timeout_<1>!\n");
}
if(print_switch == 0)
{
printf("##YES,%s\n", ctag);
}
}
void respond_fail(int client, unsigned char *ctag, int type)
{
/* <cr><lf><lf>
^^^<sid>^<date>^<time><cr><lf>
M^^<catg>^DENY<cr><lf>
^^^<error code><cr><lf>;*/
int send_ok, len;
unsigned char sendbuf[MAXDATASIZE];
memset(sendbuf, '\0', MAXDATASIZE);
get_time_fromnet();
sprintf((char *)sendbuf, "%c%c%c%c%c%c%s%c", CR, LF, LF, SP, SP, SP, conf_content.slot_u.tid, SP);
sprintf((char *)sendbuf, "%s%s%c%s%c%c", sendbuf, mcp_date, SP, mcp_time, CR, LF);
sprintf((char *)sendbuf, "%sM%c%c%s%cDENY%c%c", sendbuf, SP, SP, ctag, SP, CR, LF);
sprintf((char *)sendbuf, "%s%c%c%c%s%c%c;", sendbuf, SP, SP, SP, g_cmd_fun_ret[type], CR, LF);
len = strlen(sendbuf);
send_ok = send(client, sendbuf, len + 1, MSG_NOSIGNAL);
if(send_ok == -1)
{
/* close(client);
FD_CLR(client, &allset);
client= -1;*/
printf("timeout_<2>!\n");
}
if(print_switch == 0)
{
printf("##NO,%s\n", ctag);
}
}
void rpt_event_framing(unsigned char *event,
char slot,
int flag_na,
unsigned char *data,
int flag_sa,
unsigned char *reason)
{
unsigned char tmp[1024];
memset(tmp, '\0', 1024);
get_time_fromnet();
sprintf((char *)tmp, "%c%c%c%c%c%c%s%c", CR, LF, LF, SP, SP, SP, conf_content.slot_u.tid, SP);
sprintf((char *)tmp, "%s%s%c%s%c%c", tmp, mcp_date, SP, mcp_time, CR, LF);
sprintf((char *)tmp, "%sA%c%c%06d%cREPT%cEVNT%c%s%c%c", tmp, SP, SP, atag, SP, SP, SP, event, CR, LF);
if((event[0]=='C')&&(event[1]=='0')&&(event[2]=='0'))
{
if(conf_content.slot_u.fb == '0')
{
if(slot == RB1_SLOT)
sprintf(tmp,"%s%c%c%c\"%c:%s,%s,%s,%s,%s,%s,%s,%s,%s,%s,%s,%s:\\\"\\\"\"%c%c;",
tmp,SP,SP,SP,slot,((flag_na==1)?"NA":"CL"),rpt_content.slot_o.rb_ph[0],
rpt_content.slot_o.rb_ph[1],rpt_content.slot_o.rb_ph[2],rpt_content.slot_o.rb_ph[3],rpt_content.slot_p.rb_ph[4],rpt_content.slot_o.rb_ph[4],
rpt_content.slot_p.rb_ph[5],rpt_content.slot_o.rb_ph[5],rpt_content.slot_o.rb_ph[6],
((flag_sa==1)?"SA":"NSA"),reason,CR,LF);
else if(slot == RB2_SLOT)
sprintf(tmp,"%s%c%c%c\"%c:%s,%s,%s,%s,%s,%s,%s,%s,%s,%s,%s,%s:\\\"\\\"\"%c%c;",
tmp,SP,SP,SP,slot,((flag_na==1)?"NA":"CL"),rpt_content.slot_p.rb_ph[0],
rpt_content.slot_p.rb_ph[1],rpt_content.slot_p.rb_ph[2],rpt_content.slot_p.rb_ph[3],rpt_content.slot_p.rb_ph[4],rpt_content.slot_o.rb_ph[4],
rpt_content.slot_p.rb_ph[5],rpt_content.slot_o.rb_ph[5],rpt_content.slot_p.rb_ph[6],
((flag_sa==1)?"SA":"NSA"),reason,CR,LF);
}
else
{
if(slot == RB1_SLOT)
sprintf(tmp,"%s%c%c%c\"%c:%s,%s,%s,%s,%s,%s,%s,,,%s,%s,%s:\\\"\\\"\"%c%c;",
tmp,SP,SP,SP,slot,((flag_na==1)?"NA":"CL"),rpt_content.slot_o.rb_ph[0],
rpt_content.slot_o.rb_ph[1],rpt_content.slot_o.rb_ph[2],rpt_content.slot_o.rb_ph[3],rpt_content.slot_o.rb_ph[4],
rpt_content.slot_o.rb_ph[5],rpt_content.slot_o.rb_ph[6],
((flag_sa==1)?"SA":"NSA"),reason,CR,LF);
else if(slot == RB2_SLOT)
sprintf(tmp,"%s%c%c%c\"%c:%s,%s,%s,%s,%s,%s,%s,,,%s,%s,%s:\\\"\\\"\"%c%c;",
tmp,SP,SP,SP,slot,((flag_na==1)?"NA":"CL"),rpt_content.slot_p.rb_ph[0],
rpt_content.slot_p.rb_ph[1],rpt_content.slot_p.rb_ph[2],rpt_content.slot_p.rb_ph[3],rpt_content.slot_p.rb_ph[4],
rpt_content.slot_p.rb_ph[5],rpt_content.slot_p.rb_ph[6],
((flag_sa==1)?"SA":"NSA"),reason,CR,LF);
}
//printf("send client data,%s\n",tmp);
}
else
sprintf((char *)tmp, "%s%c%c%c\"%c:%s,%s,%s,%s:\\\"\\\"\"%c%c;",
tmp, SP, SP, SP, slot, ((flag_na == 1) ? "NA" : "CL"), data, ((flag_sa == 1) ? "SA" : "NSA"), reason, CR, LF);
sendto_all_client(tmp);
atag++;
if(atag >= 0xffff)
{
atag = 0;
}
}
/*
<cr><lf><lf>
^^^<sid>^<date>^<time><cr><lf>
<almcde>^<atag>^REPT^EVNT^<event><cr><lf>
^^^”<aid>:<ntfcncde>,<condtype>,<serveff>,<reason>:\”<conddescr>\””<cr><lf>
;
<ntfcncde>::=NA|CL
<serveff>::=SA|NSA
LT00000000000002 2011-11-19 10:27:41
A 003366 REPT EVNT C91
"l:NA,,NSA,AUTO:\"\""
;
*/
void rpt_alm_framing(char slot, unsigned char *ntfcncde,
unsigned char *almcde, unsigned char *alm)
{
/*<cr><lf><lf>
^^^<sid>^<date>^<time><cr><lf>
<almcde>^<atag>^REPT^ALRM<cr><lf>
^^^”<aid>:<ntfcncde>,<condtype>,<serveff>:\”<conddescr>\””<cr><lf>
;*/
/*<almcde>::=*C|**|*^|A^ 对应的<ctfcncde>格式为:
<ntfcncde>::=CR|MJ|MN|CL
分别表示严重告警、主要告警、次要告警及告警清除信息;*/
/*
LT00000000000002 2011-11-19 10:36:34
* 003412 REPT ALRM
"g:MN,co,NSA:\"\""
;
*/
unsigned char tmp[1024];
memset(tmp, '\0', 1024);
get_time_fromnet();
sprintf((char *)tmp, "%c%c%c%c%c%c%s%c", CR, LF, LF, SP, SP, SP, conf_content.slot_u.tid, SP);
sprintf((char *)tmp, "%s%s%c%s%c%c", tmp, mcp_date, SP, mcp_time, CR, LF);
sprintf((char *)tmp, "%s%s%c%06d%cREPT%cALRM%c%c%c", tmp, almcde, SP, atag, SP, SP, SP, CR, LF);
sprintf((char *)tmp, "%s%c%c%c\"%c:%s,%s,NSA:\\\"\\\"\"%c%c;",
tmp, SP, SP, SP, slot, ntfcncde, alm, CR, LF);
sendto_all_client(tmp);
atag++;
if(atag >= 0xffff)
{
atag = 0;
}
}
void send_online_framing(int num, char type)
{
/*<cr><lf><lf>
^^^<sid>^<date>^<time><cr><lf>
<almcde>^<atag>^REPT^EVNT^<event><cr><lf>
^^^”<aid>:<ntfcncde>,<condtype>,<serveff>,<reason>:\”<conddescr>\””<cr><lf>
;
LT00000000000002 2011-11-19 10:27:45
A 003367 REPT EVNT C91
"m:NA,O,NSA,AUTO:\"\""
;
*/
// unsigned char *tmp=(unsigned char *) malloc ( sizeof(unsigned char) * 1024 );
unsigned char tmp[1024];
if(num > 24 || num < 0)
{
return ;
}
memset(tmp, '\0', 1024);
get_time_fromnet();
sprintf((char *)tmp, "%c%c%c%c%c%c%s%c", CR, LF, LF, SP, SP, SP, conf_content.slot_u.tid, SP);
sprintf((char *)tmp, "%s%s%c%s%c%c", tmp, mcp_date, SP, mcp_time, CR, LF);
sprintf((char *)tmp, "%sA%c%c%06d%cREPT%cEVNT%cC91%c%c", tmp, SP, SP, atag, SP, SP, SP, CR, LF);
sprintf((char *)tmp, "%s%c%c%c\"%c:NA,%c,NSA,AUTO:\\\"\\\"\"%c%c;",
tmp, SP, SP, SP, (char)(num + 0x61), type, CR, LF);
sendto_all_client(tmp);
atag++;
if(atag >= 0xffff)
{
atag = 0;
}
// free(tmp);
// tmp=NULL;
}
void sendto_all_client(unsigned char *buf)
{
int count, len, i, send_ok;
int client_num[MAX_CLIENT];
len = strlen_r(buf, ';') + 1;
count = judge_client_online(client_num);
for(i = 0; i < count; i++)
{
send_ok = send(client_fd[client_num[i]], buf, len, MSG_NOSIGNAL);
//printf("\n\n%s SEND: %s\n\n", __func__, buf);
if(send_ok == -1)
{
printf("-----------------timeout!\n");
}
}
}
void sendtodown(unsigned char *buf, unsigned char slot)
{
struct mymsgbuf msgbuffer;
int msglen;
memset(&msgbuffer, '\0', sizeof(struct mymsgbuf));
msgbuffer.msgtype = 4;
msgbuffer.slot = slot - 0x60;
strcpy(msgbuffer.ctrlstring, buf);
msgbuffer.len = strlen(msgbuffer.ctrlstring);
memcpy(msgbuffer.ctag, "000000", 6);
msglen = sizeof(msgbuffer) - 4;
if((msgsnd (qid, &msgbuffer, msglen, 0)) == -1)
{
perror("msgget error<1>!\n");
printf("errno=%d\n", errno);
}
if(print_switch == 0)
{
printf("UPMSG>%s<>%d<>%s<\n", msgbuffer.ctrlstring, msgbuffer.len, msgbuffer.ctag);
}
}
void sendtodown_cli(unsigned char *buf, unsigned char slot, unsigned char *ctag)
{
struct mymsgbuf msgbuffer;
int msglen;
memset(&msgbuffer, '\0', sizeof(struct mymsgbuf));
msgbuffer.msgtype = 4;
msgbuffer.slot = slot - 0x60;
strcpy(msgbuffer.ctrlstring, buf);
msgbuffer.len = strlen(msgbuffer.ctrlstring);
memcpy (msgbuffer.ctag, ctag, 6);
msglen = sizeof(msgbuffer) - 4;
if((msgsnd (qid, &msgbuffer, msglen, 0)) == -1)
{
perror ("msgget error<2>!\n");
printf("errno=%d\n", errno);
}
if(print_switch == 0)
{
printf("UPMSG>%s<>%d<>%s<\n", msgbuffer.ctrlstring, msgbuffer.len, msgbuffer.ctag);
}
}
/*
1 成功
0 失败
*/
int ext_issue_cmd(u8_t *buf, u16_t len, u8_t slot, u8_t *ctag)
{
struct mymsgbuf msgbuffer;
int msglen;
memset(&msgbuffer, '\0', sizeof(struct mymsgbuf));
msgbuffer.msgtype = 4;
memcpy(msgbuffer.ctrlstring, buf, len);
msgbuffer.len = len;
memcpy(msgbuffer.ctag, ctag, 6);
msglen = sizeof(msgbuffer) - 4;
if( (EXT_DRV == slot_type[12]) &&
(slot >= EXT_1_OUT_LOWER && slot <= EXT_1_OUT_UPPER) &&
(1 == gExtCtx.drv[0].drvPR[0]) )
{
msgbuffer.slot = 13;
if(-1 == (msgsnd(qid, &msgbuffer, msglen, 0)))
{
return 0;
}
}
else if((EXT_DRV == slot_type[12]) &&
(slot >= EXT_2_OUT_LOWER && slot <= EXT_2_OUT_UPPER) &&
(1 == gExtCtx.drv[0].drvPR[1]) )
{
msgbuffer.slot = 13;
if(-1 == (msgsnd(qid, &msgbuffer, msglen, 0)))
{
return 0;
}
}
else if((EXT_DRV == slot_type[12]) &&
(slot >= EXT_3_OUT_LOWER && slot <= EXT_3_OUT_UPPER && slot != ':' && slot != ';') &&
(1 == gExtCtx.drv[0].drvPR[2]) )
{
msgbuffer.slot = 13;
if(-1 == (msgsnd(qid, &msgbuffer, msglen, 0)))
{
return 0;
}
}
else if((EXT_DRV == slot_type[13]) &&
(slot >= EXT_1_OUT_LOWER && slot <= EXT_1_OUT_UPPER) &&
(1 == gExtCtx.drv[1].drvPR[0]) )
{
msgbuffer.slot = 14;
if(-1 == (msgsnd(qid, &msgbuffer, msglen, 0)))
{
return 0;
}
}
else if((EXT_DRV == slot_type[13]) &&
(slot >= EXT_2_OUT_LOWER && slot <= EXT_2_OUT_UPPER) &&
(1 == gExtCtx.drv[1].drvPR[1]) )
{
msgbuffer.slot = 14;
if(-1 == (msgsnd(qid, &msgbuffer, msglen, 0)))
{
return 0;
}
}
else if((EXT_DRV == slot_type[13]) &&
(slot >= EXT_3_OUT_LOWER && slot <= EXT_3_OUT_UPPER && slot != ':' && slot != ';') &&
(1 == gExtCtx.drv[1].drvPR[2]) )
{
msgbuffer.slot = 14;
if(-1 == (msgsnd(qid, &msgbuffer, msglen, 0)))
{
return 0;
}
}
else
{
//do nothing
}
if(print_switch == 0)
{
printf("UPMSG>%s<>%d<>%s<\n", msgbuffer.ctrlstring, msgbuffer.len, msgbuffer.ctag);
}
return 1;
}
int get_freq_lev(int lev)//快捕频率的时候 降一等级
{
switch (lev)
{
case 0x00:
lev = 0x00;
break;
case 0x02:
lev = 0x04;
break;
case 0x04:
lev = 0x08;
break;
case 0x08:
lev = 0x0b;
break;
case 0x0b:
lev = 0x0f;
break;
case 0x0f:
lev = 0x0f;
break;
default:
lev = 0x0f;
break;
}
return lev;
}
int get_out_now_lev(unsigned char *parg)
{
int lev;
if(strncmp1(parg, "00", 2) == 0)
{
lev = 0;
}
else if(strncmp1(parg, "02", 2) == 0)
{
lev = 0x02;
}
else if(strncmp1(parg, "04", 2) == 0)
{
lev = 0x04;
}
else if(strncmp1(parg, "08", 2) == 0)
{
lev = 0x08;
}
else if(strncmp1(parg, "0B", 2) == 0)
{
lev = 0x0b;
}
else if(strncmp1(parg, "0F", 2) == 0)
{
lev = 0x0f;
}
else
{
lev = 0x0f;
}
return lev;
}
void get_out_now_lev_char(int lev )
{
if(lev == 0x00)
{
memcpy(conf_content.slot_u.tl, "00", 2);
}
else if(lev == 0x02)
{
memcpy(conf_content.slot_u.tl, "02", 2);
}
else if(lev == 0x04)
{
memcpy(conf_content.slot_u.tl, "04", 2);
}
else if(lev == 0x08)
{
memcpy(conf_content.slot_u.tl, "08", 2);
}
else if(lev == 0x0b)
{
memcpy(conf_content.slot_u.tl, "0B", 2);
}
else if(lev == 0x0f)
{
memcpy(conf_content.slot_u.tl, "0F", 2);
}
else if(lev == 0xff)
{
memcpy(conf_content.slot_u.tl, "FF", 2);
}
else
{
memcpy(conf_content.slot_u.tl, "00", 2);
}
}
void get_out_lev(int num, int flag)
{
int flag_lev = 0;
//int tmp_lev=0;
int c_port = 0;
int i;
int tmp1, tmp2;
unsigned char alm[3];
unsigned short alm_num = 47;
unsigned char ntfcncde[3];
unsigned char almcde[3];
unsigned char rled_en = 0;
unsigned char yled_en = 0;
if(strncmp1(rpt_content.slot_o.rb_msmode, "MAIN", 4) == 0)
{
if(strncmp1(rpt_content.slot_o.rb_trmode, "FREE", 4) == 0)
{
flag_lev = 0x0f;
rled_en = 0x01;
}
else if(strncmp1(rpt_content.slot_o.rb_trmode, "HOLD", 4) == 0)
{
yled_en = 0x01;
if(slot_type[14] == 'K')
{
flag_lev = 0x04;
}
else if(slot_type[14] == 'R')
{
flag_lev = 0x0b;
}
}
else if((strncmp1(rpt_content.slot_o.rb_trmode, "FATR", 4) == 0)
|| (strncmp1(rpt_content.slot_o.rb_trmode, "TRCK", 4) == 0))
{
yled_en = 0x01;
i = rpt_content.slot_o.rb_ref - 0x31;
if((i >= 0) && (i < 4))
{
if(strncmp1(rpt_content.slot_o.rb_trmode, "FATR", 4) == 0)
{
flag_lev = 0x04;
}
else
{
flag_lev = 0x02;
}
}
else if((i > 3) && (i < 8))
{
if(conf_content.slot_u.fb == '0')
{
if(i == 4)/* INP-5、INP-6,分别代表RB1、RB2盘的2Mhz输入信号
INP-7、INP-8,分别代表RB1、RB2盘的2Mbit输入信号
2hz下发的时钟等级已知,只需转发SSM字节
*/
{
//读取寄存器 获取TL
}
else if(i == 5)
{
/*NULL*/
flag_lev = get_ref_tl(rpt_content.slot_o.rb_tl_2mhz);
if(strncmp1(rpt_content.slot_o.rb_trmode, "FATR", 4) == 0)
{
flag_lev = get_freq_lev(flag_lev);
}
}
else if(i == 6)
{
}
else if(i == 7)
{
/*NULL*/
flag_lev = get_ref_tl(rpt_content.slot_o.rb_tl);
if(strncmp1(rpt_content.slot_o.rb_trmode, "FATR", 4) == 0)
{
flag_lev = get_freq_lev(flag_lev);
}
}
}
else if (conf_content.slot_u.fb == '1') //后面板
{
if(i == 4) //ref1
{
if((rpt_content.slot[0].ref_mod1[2] > '0') && (rpt_content.slot[0].ref_mod1[2] < '9'))
{
c_port = rpt_content.slot[0].ref_mod1[2] - 0x31;
flag_lev = get_ref_tl(rpt_content.slot[0].ref_tl[c_port]);
if(strncmp1(rpt_content.slot_o.rb_trmode, "FATR", 4) == 0)
{
flag_lev = get_freq_lev(flag_lev);
}
if('1' == conf_content.slot_u.out_ssm_en)
{
tmp1 = flag_lev;
if(tmp1 == 0)
{
tmp1 = 16;
}
tmp2 = conf_content.slot_u.out_ssm_oth;
if(tmp2 == 0)
{
tmp2 = 16;
}
if(tmp1 > tmp2)
{
ssm_alm_curr = 1;// 1表示有告警
}
else
{
ssm_alm_curr = 0;
}
//SSM门限 告警产生
if(0 == ssm_alm_last && 1 == ssm_alm_curr)
{
ssm_alm_last = ssm_alm_curr;
alm[0] = ((alm_num & 0x0f) | 0x60);
alm[1] = (((alm_num & 0x30) >> 3) | 0x69);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_MN, 2);
ntfcncde[2] = '\0';
memcpy(almcde, TYPE_MN_A, 2);
almcde[2] = '\0';
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
//SSM门限 告警清除
if(1 == ssm_alm_last && 0 == ssm_alm_curr)
{
ssm_alm_last = ssm_alm_curr;
alm[0] = ((alm_num & 0x0f) | 0x60);
alm[1] = (((alm_num & 0x30) >> 3) | 0x68);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_CL, 2);
ntfcncde[2] = '\0';
memcpy(almcde, TYPE_CL_A, 2);
almcde[2] = '\0';
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
}
else
{
flag_lev = 0xff;
//SSM闭塞时,如果已经产生SSM门限告警,需要清除此告警
if(1 == ssm_alm_curr)
{
ssm_alm_curr = 0;
alm[0] = ((alm_num & 0x0f) | 0x60);
alm[1] = (((alm_num & 0x30) >> 3) | 0x68);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_CL, 2);
ntfcncde[2] = '\0';
memcpy(almcde, TYPE_CL_A, 2);
almcde[2] = '\0';
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
}
}
}
else if(i == 5) //ref2
{
if((rpt_content.slot[1].ref_mod1[2] > '0') && (rpt_content.slot[1].ref_mod1[2] < '9'))
{
c_port = rpt_content.slot[1].ref_mod1[2] - 0x31;
flag_lev = get_ref_tl(rpt_content.slot[1].ref_tl[c_port]);
if(strncmp1(rpt_content.slot_o.rb_trmode, "FATR", 4) == 0)
{
flag_lev = get_freq_lev(flag_lev);
}
if('1' == conf_content.slot_u.out_ssm_en)
{
tmp1 = flag_lev;
if(tmp1 == 0)
{
tmp1 = 16;
}
tmp2 = conf_content.slot_u.out_ssm_oth;
if(tmp2 == 0)
{
tmp2 = 16;
}
if(tmp1 > tmp2)
{
ssm_alm_curr = 1;// 1表示有告警
}
else
{
ssm_alm_curr = 0;
}
//SSM门限 告警产生
if(0 == ssm_alm_last && 1 == ssm_alm_curr)
{
ssm_alm_last = ssm_alm_curr;
alm[0] = ((alm_num & 0x0f) | 0x60);
alm[1] = (((alm_num & 0x30) >> 3) | 0x69);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_MN, 2);
ntfcncde[2] = '\0';
memcpy(almcde, TYPE_MN_A, 2);
almcde[2] = '\0';
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
//SSM门限 告警清除
if(1 == ssm_alm_last && 0 == ssm_alm_curr)
{
ssm_alm_last = ssm_alm_curr;
alm[0] = ((alm_num & 0x0f) | 0x60);
alm[1] = (((alm_num & 0x30) >> 3) | 0x68);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_CL, 2);
ntfcncde[2] = '\0';
memcpy(almcde, TYPE_CL_A, 2);
almcde[2] = '\0';
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
}
else
{
flag_lev = 0xff;
//SSM闭塞时,如果已经产生SSM门限告警,需要清除此告警
if(1 == ssm_alm_curr)
{
ssm_alm_curr = 0;
alm[0] = ((alm_num & 0x0f) | 0x60);
alm[1] = (((alm_num & 0x30) >> 3) | 0x68);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_CL, 2);
ntfcncde[2] = '\0';
memcpy(almcde, TYPE_CL_A, 2);
almcde[2] = '\0';
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
}
}
}
}
}
else
{
printf("ref change %c\n", rpt_content.slot_o.rb_ref);
return;
}
}
else
{
printf("clock status unknow %s\n",rpt_content.slot_o.rb_trmode);
return;
}
}
else if(strncmp1(rpt_content.slot_p.rb_msmode, "MAIN", 4) == 0)
{
if(strncmp1(rpt_content.slot_p.rb_trmode, "FREE", 4) == 0)
{
rled_en = 0x01;
flag_lev = 0x0f;
}
else if(strncmp1(rpt_content.slot_p.rb_trmode, "HOLD", 4) == 0)
{
yled_en = 0x01;
if(slot_type[15] == 'K')
{
flag_lev = 0x04;
}
else if(slot_type[15] == 'R')
{
flag_lev = 0x0b;
}
}
else if((strncmp1(rpt_content.slot_p.rb_trmode, "FATR", 4) == 0)
|| (strncmp1(rpt_content.slot_p.rb_trmode, "TRCK", 4) == 0))
{
yled_en = 0x01;
i = rpt_content.slot_p.rb_ref - 0x31;
if((i >= 0) && (i < 4)) //时间
{
if(strncmp1(rpt_content.slot_p.rb_trmode, "FATR", 4) == 0)
{
flag_lev = 0x04;
}
else
{
flag_lev = 0x02;
}
}
else if((i > 3) && (i < 8)) //pinglv
{
if(conf_content.slot_u.fb == '0')
{
if(i == 4)
{
flag_lev = get_ref_tl(rpt_content.slot_p.rb_tl_2mhz);
if(strncmp1(rpt_content.slot_p.rb_trmode, "FATR", 4) == 0)
{
flag_lev = get_freq_lev(flag_lev);
}
}
else if(i == 5)
{
}
else if(i == 6)
{
flag_lev = get_ref_tl(rpt_content.slot_p.rb_tl);
if(strncmp1(rpt_content.slot_p.rb_trmode, "FATR", 4) == 0)
{
flag_lev = get_freq_lev(flag_lev);
}
}
else if(i == 7)
{
}
}
else if(conf_content.slot_u.fb == '1') //后面板
{
if(i == 4) //ref1
{
if((rpt_content.slot[0].ref_mod1[2] > '0') && (rpt_content.slot[0].ref_mod1[2] < '9'))
{
c_port = rpt_content.slot[0].ref_mod1[2] - 0x31;
flag_lev = get_ref_tl(rpt_content.slot[0].ref_tl[c_port]);
if(strncmp1(rpt_content.slot_p.rb_trmode, "FATR", 4) == 0)
{
flag_lev = get_freq_lev(flag_lev);
}
if('1' == conf_content.slot_u.out_ssm_en)
{
tmp1 = flag_lev;
if(tmp1 == 0)
{
tmp1 = 16;
}
tmp2 = conf_content.slot_u.out_ssm_oth;
if(tmp2 == 0)
{
tmp2 = 16;
}
if(tmp1 > tmp2)
{
ssm_alm_curr = 1;// 1表示有告警
}
else
{
ssm_alm_curr = 0;
}
//SSM门限 告警产生
if(0 == ssm_alm_last && 1 == ssm_alm_curr)
{
ssm_alm_last = ssm_alm_curr;
alm[0] = ((alm_num & 0x0f) | 0x60);
alm[1] = (((alm_num & 0x30) >> 3) | 0x69);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_MN, 2);
ntfcncde[2] = '\0';
memcpy(almcde, TYPE_MN_A, 2);
almcde[2] = '\0';
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
//SSM门限 告警清除
if(1 == ssm_alm_last && 0 == ssm_alm_curr)
{
ssm_alm_last = ssm_alm_curr;
alm[0] = ((alm_num & 0x0f) | 0x60);
alm[1] = (((alm_num & 0x30) >> 3) | 0x68);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_CL, 2);
ntfcncde[2] = '\0';
memcpy(almcde, TYPE_CL_A, 2);
almcde[2] = '\0';
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
}
else
{
flag_lev = 0xff;
//SSM闭塞时,如果已经产生SSM门限告警,需要清除此告警
if(1 == ssm_alm_curr)
{
ssm_alm_curr = 0;
alm[0] = ((alm_num & 0x0f) | 0x60);
alm[1] = (((alm_num & 0x30) >> 3) | 0x68);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_CL, 2);
ntfcncde[2] = '\0';
memcpy(almcde, TYPE_CL_A, 2);
almcde[2] = '\0';
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
}
}
}
else if(i == 5) //ref2
{
if((rpt_content.slot[1].ref_mod1[2] > '0') && (rpt_content.slot[1].ref_mod1[2] < '9'))
{
c_port = rpt_content.slot[1].ref_mod1[2] - 0x31;
flag_lev = get_ref_tl(rpt_content.slot[1].ref_tl[c_port]);
if(strncmp1(rpt_content.slot_p.rb_trmode, "FATR", 4) == 0)
{
flag_lev = get_freq_lev(flag_lev);
}
if('1' == conf_content.slot_u.out_ssm_en)
{
tmp1 = flag_lev;
if(tmp1 == 0)
{
tmp1 = 16;
}
tmp2 = conf_content.slot_u.out_ssm_oth;
if(tmp2 == 0)
{
tmp2 = 16;
}
if(tmp1 > tmp2)
{
ssm_alm_curr = 1;// 1表示有告警
}
else
{
ssm_alm_curr = 0;
}
//SSM门限 告警产生
if(0 == ssm_alm_last && 1 == ssm_alm_curr)
{
ssm_alm_last = ssm_alm_curr;
alm[0] = ((alm_num & 0x0f) | 0x60);
alm[1] = (((alm_num & 0x30) >> 3) | 0x69);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_MN, 2);
ntfcncde[2] = '\0';
memcpy(almcde, TYPE_MN_A, 2);
almcde[2] = '\0';
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
//SSM门限 告警清除
if(1 == ssm_alm_last && 0 == ssm_alm_curr)
{
ssm_alm_last = ssm_alm_curr;
alm[0] = ((alm_num & 0x0f) | 0x60);
alm[1] = (((alm_num & 0x30) >> 3) | 0x68);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_CL, 2);
ntfcncde[2] = '\0';
memcpy(almcde, TYPE_CL_A, 2);
almcde[2] = '\0';
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
}
else
{
flag_lev = 0xff;
//SSM闭塞时,如果已经产生SSM门限告警,需要清除此告警
if(1 == ssm_alm_curr)
{
ssm_alm_curr = 0;
alm[0] = ((alm_num & 0x0f) | 0x60);
alm[1] = (((alm_num & 0x30) >> 3) | 0x68);
alm[2] = '\0';
memcpy(ntfcncde, TYPE_CL, 2);
ntfcncde[2] = '\0';
memcpy(almcde, TYPE_CL_A, 2);
almcde[2] = '\0';
rpt_alm_framing('u', ntfcncde, almcde, alm);
}
}
}
}
}
}
else
{
printf("ref change %c\n", rpt_content.slot_p.rb_ref);
return;
}
}
else
{
printf("clock status unknow %s\n",rpt_content.slot_p.rb_trmode);
return;
}
}
else
{
return;
}
if(strncmp1(rpt_content.slot_p.rb_msmode, "STBY", 4) == 0)
{
if(strncmp1(rpt_content.slot_p.rb_trmode, "FREE", 4) == 0)
rled_en = 0x01;
else
yled_en = 0x01;
}
else if(strncmp1(rpt_content.slot_o.rb_msmode, "STBY", 4) == 0)
{
if(strncmp1(rpt_content.slot_p.rb_trmode, "FREE", 4) == 0)
rled_en = 0x01;
else
yled_en = 0x01;
}
if (rled_en == 0x01)
{
FpgaWrite(ALMControl, 0xfff9);
FpgaWrite(IndicateLED,0x0001);
}
else
{
FpgaWrite(ALMControl, 0xfffe);
FpgaWrite(IndicateLED,0x0002);
}
get_out_now_lev_char(flag_lev);
if(flag == 1) //初始化,单独对一个单盘下发
{
send_out_lev(flag_lev, num);
printf("singal out len slot=%d,lev=%d\n", num, flag_lev);
}
else
{
if(flag_lev != g_lev)
{
g_lev = flag_lev;
for(i = 0; i < 14; i++)
{
//g_lev = get_out_now_lev(rpt_content.slot[i].out_lev);
if( (slot_type[i] == 'J') ||
(slot_type[i] == 'a') ||
(slot_type[i] == 'b') ||
(slot_type[i] == 'c') ||
(slot_type[i] == 'h') ||
(slot_type[i] == 'i'))
{
send_out_lev(flag_lev, i);
printf("all out len slot=%d,lev=%d\n", i, flag_lev);
}
}
//扩展框 时钟等级
ext_ssm_cfg(conf_content.slot_u.tl);
}
}
}
void send_out_lev(int flag_lev, int num)
{
unsigned char sendbuf[SENDBUFSIZE];
memset(sendbuf, '\0', SENDBUFSIZE);
//printf("-------------in send -------lev=%02x\n",flag_lev);
switch(flag_lev)
{
case 0x00:
{
sprintf((char *)sendbuf, "SET-OUT-LEV:000000::00;");
sendtodown(sendbuf, (char)('a' + num));
break;
}
case 0x02:
{
sprintf((char *)sendbuf, "SET-OUT-LEV:000000::02;");
sendtodown(sendbuf, (char)('a' + num));
break;
}
case 0x04:
{
sprintf((char *)sendbuf, "SET-OUT-LEV:000000::04;");
sendtodown(sendbuf, (char)('a' + num));
break;
}
case 0x08:
{
sprintf((char *)sendbuf, "SET-OUT-LEV:000000::08;");
sendtodown(sendbuf, (char)('a' + num));
break;
}
case 0x0b:
{
sprintf((char *)sendbuf, "SET-OUT-LEV:000000::0B;");
sendtodown(sendbuf, (char)('a' + num));
break;
}
case 0x0f:
{
sprintf((char *)sendbuf, "SET-OUT-LEV:000000::0F;");
sendtodown(sendbuf, (char)('a' + num));
break;
}
case 0xff:
{
sprintf((char *)sendbuf, "SET-OUT-LEV:000000::FF;");
sendtodown(sendbuf, (char)('a' + num));
break;
}
default:
{
break;
}
}
}
int get_ref_tl(unsigned char c)
{
int lev = 0;
if(c == 'a')
{
lev = 0x02;
}
else if(c == 'b')
{
lev = 0x04;
}
else if(c == 'c')
{
lev = 0x08;
}
else if(c == 'd')
{
lev = 0x0b;
}
else if(c == 'e')
{
lev = 0x0f;
}
else if(c == 'f')
{
lev = 0x00;
}
else if(c == 'g')
{
lev = 0x00;
}
return lev;
}
int send_ref_lev(int num)
{
int c_port = 0;
int c_tl = 0;
int i;
char ext_ssm[3];
if((num != 0) && (num != 1))
{
return 1;
}
if(strncmp1(rpt_content.slot_o.rb_msmode, "MAIN", 4) == 0)
{
if((rpt_content.slot_o.rb_ref == '7') && ((num == 0)))
{
//先选择跟踪源 路数
if((rpt_content.slot[0].ref_mod1[2] > '0') && (rpt_content.slot[0].ref_mod1[2] < '9'))
{
c_port = rpt_content.slot[0].ref_mod1[2] - 0x31;
c_tl = get_ref_tl(rpt_content.slot[0].ref_tl[c_port]);
}
else
{
return 1;
}
}
else if((rpt_content.slot_o.rb_ref == '8') && ((num == 1)))
{
if((rpt_content.slot[1].ref_mod1[2] > '0') && (rpt_content.slot[1].ref_mod1[2] < '9'))
{
c_port = rpt_content.slot[1].ref_mod1[2] - 0x31;
c_tl = get_ref_tl(rpt_content.slot[1].ref_tl[c_port]);
}
else
{
return 1;
}
}
else
{
return 1;
}
}
else if(strncmp1(rpt_content.slot_p.rb_msmode, "MAIN", 4) == 0)
{
if((rpt_content.slot_p.rb_ref == '7') && ((num == 0)))
{
//先选择跟踪源 路数
if((rpt_content.slot[0].ref_mod1[2] > '0') && (rpt_content.slot[0].ref_mod1[2] < '9'))
{
c_port = rpt_content.slot[0].ref_mod1[2] - 0x31;
c_tl = get_ref_tl(rpt_content.slot[0].ref_tl[c_port]);
}
else
{
return 1;
}
}
else if((rpt_content.slot_p.rb_ref == '8') && ((num == 1)))
{
if((rpt_content.slot[1].ref_mod1[2] > '0') && (rpt_content.slot[1].ref_mod1[2] < '9'))
{
c_port = rpt_content.slot[1].ref_mod1[2] - 0x31;
c_tl = get_ref_tl(rpt_content.slot[1].ref_tl[c_port]);
}
else
{
return 1;
}
}
else
{
return 1;
}
}
else
{
return 1;
}
if(c_tl != g_lev) //再比较是否改变
{
g_lev = c_tl;
for(i = 0; i < 14; i++)
{
if( (slot_type[i] == 'J') ||
(slot_type[i] == 'a') ||
(slot_type[i] == 'b') ||
(slot_type[i] == 'c') ||
(slot_type[i] == 'h') ||
(slot_type[i] == 'i') )
{
send_out_lev(c_tl, i);
}
}
memset(ext_ssm, 0, 3);
if(0x00 == c_tl)
{
memcpy(ext_ssm, "00", 2);
}
else if(0x02 == c_tl)
{
memcpy(ext_ssm, "02", 2);
}
else if(0x04 == c_tl)
{
memcpy(ext_ssm, "04", 2);
}
else if(0x08 == c_tl)
{
memcpy(ext_ssm, "08", 2);
}
else if(0x0B == c_tl)
{
memcpy(ext_ssm, "0B", 2);
}
else if(0x0F == c_tl)
{
memcpy(ext_ssm, "0F", 2);
}
else if(0xFF == c_tl)
{
memcpy(ext_ssm, "FF", 2);
}
else
{
memcpy(ext_ssm, "00", 2);
}
//扩展框 时钟等级
ext_ssm_cfg(ext_ssm);
}
return 0;
}
int file_exists(char *filename)
{
return (access(filename, 0) == 0);
}
/*
int find_config()
{
struct dirent *ptr;
DIR *dir;
dir=opendir("/usr/");
while((ptr=readdir(dir))!=NULL)
{
if(ptr->d_name[0] == '.')
continue;
if(strncmp1(ptr->d_name,"LT",2)==0)
{
memset(exist_filename,0,50);
sprintf((char *)exist_filename,"/usr/%s",ptr->d_name);
closedir(dir);
return 1;
}
}
closedir(dir);
return 0;
}*/
/*
1 成功
0 失败
*/
int ext_alm_cmp(u8_t ext_sid, u8_t *ext_new_data, struct extctx *ctx)
{
u8_t ext_old_alm[16];
int i, j;
memset(ext_old_alm, 0, 16);
if((ext_sid >= EXT_1_OUT_LOWER) && (ext_sid <= EXT_1_OUT_UPPER))
{
memcpy(ext_old_alm, ctx->out[ext_sid - EXT_1_OUT_OFFSET].outAlm, 5);
if(0 != memcmp(ext_old_alm, ext_new_data, 5))
{
for(i = 0; i < 5; i++)
{
for(j = 0; j < 4; j++)
{
if( (0 == ((ext_old_alm[i])&BIT(j))) && (0 != ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('1', ext_sid - EXT_1_OUT_OFFSET + 97, 4 * i + j + 1, "**", "MJ");
//printf("ext out1 alarm happened %d\r\n",4 * i + j + 1);
}
else if( (0 != ((ext_old_alm[i])&BIT(j))) && (0 == ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('1', ext_sid - EXT_1_OUT_OFFSET + 97, 4 * i + j + 1, "A ", "CL");
//printf("ext out1 alarm clear %d\r\n",4 * i + j + 1);
}
else
{
//do nothing
}
}
}
}
}
else if((ext_sid >= EXT_2_OUT_LOWER) && (ext_sid <= EXT_2_OUT_UPPER))
{
memcpy(ext_old_alm, ctx->out[ext_sid - EXT_2_OUT_OFFSET].outAlm, 5);
if(0 != memcmp(ext_old_alm, ext_new_data, 5))
{
for(i = 0; i < 5; i++)
{
for(j = 0; j < 4; j++)
{
if( (0 == ((ext_old_alm[i])&BIT(j))) && (0 != ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('2', ext_sid - EXT_2_OUT_OFFSET - 10 + 97, 4 * i + j + 1, "**", "MJ");
//printf("ext out2 alarm happened %d\r\n",4 * i + j + 1);
}
else if( (0 != ((ext_old_alm[i])&BIT(j))) && (0 == ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('2', ext_sid - EXT_2_OUT_OFFSET - 10 + 97, 4 * i + j + 1, "A ", "CL");
//printf("ext out2 alarm clear %d\r\n",4 * i + j + 1);
}
else
{
//do nothing
}
}
}
}
}
else if((ext_sid >= EXT_3_OUT_LOWER) && (ext_sid <= EXT_3_OUT_UPPER))
{
if(ext_sid < 58)//except ':;'
{
memcpy(ext_old_alm, ctx->out[ext_sid - EXT_3_OUT_OFFSET].outAlm, 5);
if(0 != memcmp(ext_old_alm, ext_new_data, 5))
{
for(i = 0; i < 5; i++)
{
for(j = 0; j < 4; j++)
{
if( (0 == ((ext_old_alm[i])&BIT(j))) && (0 != ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('3', ext_sid - EXT_3_OUT_OFFSET - 20 + 97, 4 * i + j + 1, "**", "MJ");
//printf("ext out3 alarm happened %d\r\n",4 * i + j + 1);
}
else if( (0 != ((ext_old_alm[i])&BIT(j))) && (0 == ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('3', ext_sid - EXT_3_OUT_OFFSET - 20 + 97, 4 * i + j + 1, "A ", "CL");
//printf("ext out3 alarm clear %d\r\n",4 * i + j + 1);
}
else
{
//do nothing
}
}
}
}
}
else
{
memcpy(ext_old_alm, ctx->out[ext_sid - EXT_3_OUT_OFFSET - 2].outAlm, 5);
if(0 != memcmp(ext_old_alm, ext_new_data, 5))
{
for(i = 0; i < 5; i++)
{
for(j = 0; j < 4; j++)
{
if( (0 == ((ext_old_alm[i])&BIT(j))) && (0 != ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('3', ext_sid - EXT_3_OUT_OFFSET - 22 + 97, 4 * i + j + 1, "**", "MJ");
//printf("ext out3 alarm happened %d\r\n",4 * i + j + 1);
}
else if( (0 != ((ext_old_alm[i])&BIT(j))) && (0 == ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('3', ext_sid - EXT_3_OUT_OFFSET - 22 + 97, 4 * i + j + 1, "A ", "CL");
//printf("ext out3 alarm clear %d\r\n",4 * i + j + 1);
}
else
{
//do nothing
}
}
}
}
}
}
else if(EXT_1_MGR_PRI == ext_sid)
{
memcpy(ext_old_alm, ctx->mgr[0].mgrAlm, MGE_RALM_NUM);
if(0 != memcmp(ext_old_alm, ext_new_data, MGE_RALM_NUM))
{
for(i = 0; i < MGE_RALM_NUM; i++)
{
for(j = 0; j < 4; j++)
{
if( (0 == ((ext_old_alm[i])&BIT(j))) && (0 != ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('1', 'k', 4 * i + j + 1, "**", "MJ");
}
else if( (0 != ((ext_old_alm[i])&BIT(j))) && (0 == ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('1', 'k', 4 * i + j + 1, "A ", "CL");
}
else
{
//do nothing
}
}
}
}
}
else if(EXT_1_MGR_RSV == ext_sid)
{
memcpy(ext_old_alm, ctx->mgr[1].mgrAlm, MGE_RALM_NUM);
if(0 != memcmp(ext_old_alm, ext_new_data, MGE_RALM_NUM))
{
for(i = 0; i < MGE_RALM_NUM; i++)
{
for(j = 0; j < 4; j++)
{
if( (0 == ((ext_old_alm[i])&BIT(j))) && (0 != ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('1', 'l', 4 * i + j + 1, "**", "MJ");
}
else if( (0 != ((ext_old_alm[i])&BIT(j))) && (0 == ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('1', 'l', 4 * i + j + 1, "A ", "CL");
}
else
{
//do nothing
}
}
}
}
}
else if(EXT_2_MGR_PRI == ext_sid)
{
memcpy(ext_old_alm, ctx->mgr[2].mgrAlm, MGE_RALM_NUM);
if(0 != memcmp(ext_old_alm, ext_new_data, MGE_RALM_NUM))
{
for(i = 0; i < MGE_RALM_NUM; i++)
{
for(j = 0; j < 4; j++)
{
if( (0 == ((ext_old_alm[i])&BIT(j))) && (0 != ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('2', 'k', 4 * i + j + 1, "**", "MJ");
}
else if( (0 != ((ext_old_alm[i])&BIT(j))) && (0 == ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('2', 'k', 4 * i + j + 1, "A ", "CL");
}
else
{
//do nothing
}
}
}
}
}
else if(EXT_2_MGR_RSV == ext_sid)
{
memcpy(ext_old_alm, ctx->mgr[3].mgrAlm, MGE_RALM_NUM);
if(0 != memcmp(ext_old_alm, ext_new_data, MGE_RALM_NUM))
{
for(i = 0; i < MGE_RALM_NUM; i++)
{
for(j = 0; j < 4; j++)
{
if( (0 == ((ext_old_alm[i])&BIT(j))) && (0 != ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('2', 'l', 4 * i + j + 1, "**", "MJ");
}
else if( (0 != ((ext_old_alm[i])&BIT(j))) && (0 == ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('2', 'l', 4 * i + j + 1, "A ", "CL");
}
else
{
//do nothing
}
}
}
}
}
else if(EXT_3_MGR_PRI == ext_sid)
{
memcpy(ext_old_alm, ctx->mgr[4].mgrAlm, MGE_RALM_NUM);
if(0 != memcmp(ext_old_alm, ext_new_data, MGE_RALM_NUM))
{
for(i = 0; i < MGE_RALM_NUM; i++)
{
for(j = 0; j < 4; j++)
{
if( (0 == ((ext_old_alm[i])&BIT(j))) && (0 != ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('3', 'k', 4 * i + j + 1, "**", "MJ");
//printf("ext mgr3 alarm happened %d\r\n",4 * i + j + 1);
}
else if( (0 != ((ext_old_alm[i])&BIT(j))) && (0 == ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('3', 'k', 4 * i + j + 1, "A ", "CL");
//printf("ext mgr3 alarm clear %d\r\n",4 * i + j + 1);
}
else
{
//do nothing
}
}
}
}
}
else if(EXT_3_MGR_RSV == ext_sid)
{
memcpy(ext_old_alm, ctx->mgr[5].mgrAlm, MGE_RALM_NUM);
if(0 != memcmp(ext_old_alm, ext_new_data, MGE_RALM_NUM))
{
for(i = 0; i < MGE_RALM_NUM; i++)
{
for(j = 0; j < 4; j++)
{
if( (0 == ((ext_old_alm[i])&BIT(j))) && (0 != ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('3', 'l', 4 * i + j + 1, "**", "MJ");
//printf("ext mge3 alarm happened %d\r\n",4 * i + j + 1);
}
else if( (0 != ((ext_old_alm[i])&BIT(j))) && (0 == ((ext_new_data[i])&BIT(j))) )
{
ext_alm_evt('3', 'l', 4 * i + j + 1, "A ", "CL");
//printf("ext mge3 alarm clear %d\r\n",4 * i + j + 1);
}
else
{
//do nothing
}
}
}
}
}
else
{
return 0;
}
return 1;
}
/*
1 成功
0 失败
*/
int ext_alm_evt(u8_t eid, u8_t sid, u8_t almid, u8_t *agcc1, u8_t *agcc2)
{
u8_t sendbuf[1024];
signed char offset;
u8_t onlinesta;
offset = eid - '1';
onlinesta = gExtCtx.save.onlineSta;
if( offset > 2 || offset < 0)
{
return 0;
}
else
{
if( ((onlinesta >> offset) & 0x01) == 0)
{
return 0;
}
else
{
//lalalalala;
}
}
memset(sendbuf, 0, 1024);
get_time_fromnet();
sprintf((char *)sendbuf, "\r\n\n %s %s %s\r\n%s %06d EXT ALM\r\n \"%c,%c,%03d,%s\"\r\n;",
conf_content.slot_u.tid, mcp_date, mcp_time, agcc1, atag,
eid, sid, almid, agcc2);
sendto_all_client(sendbuf);
//printf("send ext alm %s\r\n",sendbuf);
atag++;
if(atag >= 0xffff)
{
atag = 0;
}
return 1;
}
/*
1 成功
0 失败
*/
int ext_bpp_cfg(u8_t ext_sid, u8_t *ext_new_type, struct extctx *ctx)
{
u8_t ext_old_type[15];
int i;
u8_t tmp_ctag[7];
u8_t sendbuf[256];
u8_t slotid;
memset(ext_old_type, 0, 15);
memset(tmp_ctag, 0, 7);
memcpy(tmp_ctag, "000000", 6);
if((EXT_1_MGR_PRI == ext_sid) || (EXT_1_MGR_RSV == ext_sid))
{
memcpy(ext_old_type, ctx->extBid[0], 14);
for(i = 0; i < 10; i++)
{
if( (EXT_NONE == ext_old_type[i]) &&
((EXT_OUT16 == ext_new_type[i]) || (EXT_OUT32 == ext_new_type[i]) || (EXT_OUT16S == ext_new_type[i]) || (EXT_OUT32S == ext_new_type[i])) )
{
// lev
printf("1+%d+%s\n", i + 1, ctx->save.outSsm[i]);
if(2 == strlen(ctx->save.outSsm[i]))
{
memset(sendbuf, 0, 256);
sprintf((char *)sendbuf, "SET-OUT-LEV:%c:%s::%s;",
i + EXT_1_OUT_LOWER,
tmp_ctag,
ctx->save.outSsm[i]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, i + EXT_1_OUT_LOWER, tmp_ctag);
}
//signal
printf("1+%d+%s\n", i + 1, ctx->save.outSignal[i]);
if(16 == strlen(ctx->save.outSignal[i]))
{
memset(sendbuf, 0, 256);
sprintf((char *)sendbuf, "SET-OUT-TYP:%c:%s::%s;",
i + EXT_1_OUT_LOWER,
tmp_ctag,
ctx->save.outSignal[i]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, i + EXT_1_OUT_LOWER, tmp_ctag);
}
//mod
printf("1+%d+%s\n", i + 1, ctx->save.outPR[i]);
if('0' == ctx->save.outPR[i][0] || '1' == ctx->save.outPR[i][0])
{
memset(sendbuf, 0, 256);
sprintf((char *)sendbuf, "SET-OUT-MOD:%c:%s::%c;",
i + EXT_1_OUT_LOWER,
tmp_ctag,
ctx->save.outPR[i][0]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, i + EXT_1_OUT_LOWER, tmp_ctag);
}
}
}
}
else if((EXT_2_MGR_PRI == ext_sid) || (EXT_2_MGR_RSV == ext_sid))
{
memcpy(ext_old_type, ctx->extBid[1], 14);
for(i = 0; i < 10; i++)
{
if( (EXT_NONE == ext_old_type[i]) &&
((EXT_OUT16 == ext_new_type[i]) || (EXT_OUT32 == ext_new_type[i]) || (EXT_OUT16S == ext_new_type[i]) || (EXT_OUT32S == ext_new_type[i])) )
{
//lev
printf("2+%d+%s\n", i + 1, ctx->save.outSsm[10 + i]);
if(2 == strlen(ctx->save.outSsm[10 + i]))
{
memset(sendbuf, 0, 256);
sprintf((char *)sendbuf, "SET-OUT-LEV:%c:%s::%s;",
i + EXT_2_OUT_LOWER,
tmp_ctag,
ctx->save.outSsm[10 + i]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, i + EXT_2_OUT_LOWER, tmp_ctag);
}
//signal
printf("2+%d+%s\n", i + 1, ctx->save.outSignal[10 + i]);
if(16 == strlen(ctx->save.outSignal[10 + i]))
{
memset(sendbuf, 0, 256);
sprintf((char *)sendbuf, "SET-OUT-TYP:%c:%s::%s;",
i + EXT_2_OUT_LOWER,
tmp_ctag,
ctx->save.outSignal[10 + i]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, i + EXT_2_OUT_LOWER, tmp_ctag);
}
//mod
printf("2+%d+%s\n", i + 1, ctx->save.outPR[10 + i]);
if('0' == ctx->save.outPR[10 + i][0] || '1' == ctx->save.outPR[10 + i][0])
{
memset(sendbuf, 0, 256);
sprintf((char *)sendbuf, "SET-OUT-MOD:%c:%s::%c;",
i + EXT_2_OUT_LOWER,
tmp_ctag,
ctx->save.outPR[10 + i][0]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, i + EXT_2_OUT_LOWER, tmp_ctag);
}
}
}
}
else if((EXT_3_MGR_PRI == ext_sid) || (EXT_3_MGR_RSV == ext_sid))
{
memcpy(ext_old_type, ctx->extBid[2], 14);
for(i = 0; i < 10; i++)
{
if( (EXT_NONE == ext_old_type[i]) &&
((EXT_OUT16 == ext_new_type[i]) || (EXT_OUT32 == ext_new_type[i]) || (EXT_OUT16S == ext_new_type[i]) || (EXT_OUT32S == ext_new_type[i])) )
{
if((i + EXT_3_OUT_LOWER) >= ':')//except ':;'
{
slotid = i + EXT_3_OUT_LOWER + 2;
}
else
{
slotid = i + EXT_3_OUT_LOWER;
}
//lev
printf("3+%d+%s\n", i + 1, ctx->save.outSsm[20 + i]);
if(2 == strlen(ctx->save.outSsm[20 + i]))
{
memset(sendbuf, 0, 256);
sprintf((char *)sendbuf, "SET-OUT-LEV:%c:%s::%s;",
slotid,
tmp_ctag,
ctx->save.outSsm[20 + i]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, slotid, tmp_ctag);
}
//signal
printf("3+%d+%s\n", i + 1, ctx->save.outSignal[20 + i]);
if(16 == strlen(ctx->save.outSignal[20 + i]))
{
memset(sendbuf, 0, 256);
sprintf((char *)sendbuf, "SET-OUT-TYP:%c:%s::%s;",
slotid,
tmp_ctag,
ctx->save.outSignal[20 + i]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, slotid, tmp_ctag);
}
//mod
printf("3+%d+%s\n", i + 1, ctx->save.outPR[20 + i]);
if('0' == ctx->save.outPR[20 + i][0] || '1' == ctx->save.outPR[20 + i][0])
{
memset(sendbuf, 0, 256);
sprintf((char *)sendbuf, "SET-OUT-MOD:%c:%s::%c;",
slotid,
tmp_ctag,
ctx->save.outPR[20 + i][0]);
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, slotid, tmp_ctag);
}
}
}
}
else
{
return 0;
}
return 1;
}
int ethn_down(const char *networkcard)
{
int sock_fd;
struct ifreq ifr;
if ( ( sock_fd = socket( AF_INET, SOCK_STREAM, 0 ) ) < 0 )
{
close(sock_fd);
return (0);
}
memset( &ifr, 0, sizeof(struct ifreq) );
strncpy( ifr.ifr_name, networkcard, IFNAMSIZ );
if (ioctl(sock_fd, SIOCGIFFLAGS, &ifr) < 0)
{
close(sock_fd);
return (0);
}
ifr.ifr_flags &= (~IFF_UP);
if ( ioctl ( sock_fd, SIOCSIFFLAGS, &ifr) < 0 )
{
close(sock_fd);
return (0);
}
close(sock_fd);
return (1);
}
int ethn_up(const char *networkcard)
{
int sock_fd;
struct ifreq ifr;
if ( ( sock_fd = socket( AF_INET, SOCK_STREAM, 0 ) ) < 0 )
{
close(sock_fd);
return (0);
}
memset( &ifr, 0, sizeof(struct ifreq) );
strncpy( ifr.ifr_name, networkcard, IFNAMSIZ );
if (ioctl(sock_fd, SIOCGIFFLAGS, &ifr) < 0)
{
close(sock_fd);
return (0);
}
ifr.ifr_flags |= (IFF_UP | IFF_RUNNING);
if ( ioctl ( sock_fd, SIOCSIFFLAGS, &ifr) < 0 )
{
close(sock_fd);
return (0);
}
close(sock_fd);
return (1);
}
int mcp_get_gateway( char *local_gateway )
{
char buf[1024];
int pl = 0, ph = 0;
int i;
FILE *readfp;
memset( buf, 0, sizeof(buf) );
readfp = popen( "route -n", "r" );
if ( NULL == readfp )
{
printf("route -n is NULL\n");
pclose( readfp );
return(0);
}
if ( 0 == fread( buf, sizeof(char), sizeof(buf), readfp) )
{
pclose( readfp );
return (0);
}
for( i = 0; i < 1024; i++ )
{
if ( buf[i] == 'U' && buf[i + 1] == 'G' && (i + 1) < 1024 )
{
pl = i;
break;
}
}
if( pl == 0 )
{
pclose( readfp );
return(0);
}
while ( buf[pl] > '9' || buf[pl] < '0' )
{
pl--;
}
while ( (buf[pl] >= '0' && buf[pl] <= '9') || buf[pl] == '.')
{
pl--;
}
while ( buf[pl] > '9' || buf[pl] < '0' )
{
pl--;
}
buf[pl + 1] = '\0';
for ( i = pl; ((buf[i] >= '0' && buf[i] <= '9') || buf[i] == '.'); i--);
ph = i + 1;
strcpy(local_gateway, &buf[ph]);
//printf("<mcp_get_gateway>:%s\n",local_gateway);
pclose( readfp );
return (1);
}
int mcp_set_gateway( char *networkcard, char *old_gateway, char *new_gateway )
{
int sock_fd = -1;
struct rtentry rt;
struct sockaddr rtsockaddr;
struct sockaddr_in *sin = NULL;
if ( (sock_fd = socket(AF_INET, SOCK_STREAM, 0) ) < 0 )
{
close(sock_fd);
return(0);
}
memset(&rt, 0, sizeof(struct rtentry));
memset(&rtsockaddr, 0, sizeof(struct sockaddr));
sin = (struct sockaddr_in *)&rtsockaddr;
sin->sin_family = AF_INET;
sin->sin_addr.s_addr = INADDR_ANY;
rt.rt_dst = rtsockaddr; //set the destination address to '0.0.0.0'
rt.rt_genmask = rtsockaddr; //set the netmask to '0.0.0.0'
//Fill in the other fields.
rt.rt_flags = (RTF_UP | RTF_GATEWAY);
rt.rt_metric = 0;
rt.rt_dev = networkcard;
//delete the current default gateway.
sin->sin_addr.s_addr = inet_addr(old_gateway);
rt.rt_gateway = rtsockaddr;
if (ioctl(sock_fd, SIOCDELRT, &rt) < 0)
{
if( errno != ESRCH)
{
close(sock_fd);
return(0);
}
}
//set the new default gateway.
sin->sin_addr.s_addr = inet_addr(new_gateway);
rt.rt_gateway = rtsockaddr;
if (ioctl(sock_fd, SIOCADDRT, &rt) < 0)
{
close(sock_fd);
return(0);
}
close(sock_fd);
return(1);
}
void mcpd_reset_network_card(void)
{
int retval = 0;
unsigned char gateway[16];
memset(gateway, 0, sizeof(gateway));
retval = mcp_get_gateway(gateway);
if( 1 == retval )
{
printf("reset_network_card: 1\n");
if ( 1 == ethn_down("eth0"))
{
printf("eth0 down success!!!\n");
while ( 0 == ethn_up("eth0") )
{
printf("eth0 up failure!!!\n");
}
printf("eth0 up success!!!\n");
mcp_set_gateway(TCP_NETWORK_CARD, gateway, gateway);
}
else
{
if(print_switch == 0)
{
printf("eth0 down failure!!!\n");
}
}
}
else//2014-1-24添加else语句,未获取成功时,使用flash中保存的网关
{
printf("reset_network_card: 0\n");
memcpy(gateway, conf_content.slot_u.gate, 16);
if ( 1 == ethn_down("eth0"))
{
printf("eth0 down success!!!\n");
while ( 0 == ethn_up("eth0") )
{
printf("eth0 up failure!!!\n");
}
printf("eth0 up success!!!\n");
mcp_set_gateway(TCP_NETWORK_CARD, gateway, gateway);
}
else
{
if(print_switch == 0)
{
printf("eth0 down failure!!!\n");
}
}
}
}
/*
1 成功
0 失败
*/
int ext_drv_mgr_evt(struct extctx *ctx)
{
u8_t sendbuf[1024];
u8_t aid;
memset(ctx->new_drv_mgr_sta, 0, 32);
sprintf((char *) ctx->new_drv_mgr_sta,
"%d%d%d%d%d%d,%d%d%d%d%d%d",
ctx->drv[0].drvPR[0], ctx->drv[1].drvPR[0],
ctx->drv[0].drvPR[1], ctx->drv[1].drvPR[1],
ctx->drv[0].drvPR[2], ctx->drv[1].drvPR[2],
ctx->mgr[0].mgrPR, ctx->mgr[1].mgrPR,
ctx->mgr[2].mgrPR, ctx->mgr[3].mgrPR,
ctx->mgr[4].mgrPR, ctx->mgr[5].mgrPR );
if(0 != strcmp(ctx->old_drv_mgr_sta, ctx->new_drv_mgr_sta))
{
memcpy(ctx->old_drv_mgr_sta, ctx->new_drv_mgr_sta, 13);
memset(sendbuf, 0, 1024);
get_time_fromnet();
if('d' == slot_type[12])
{
aid = 'm';
}
else if('d' == slot_type[13])
{
aid = 'n';
}
else
{
aid = 'm';
}
sprintf((char *)sendbuf, "\r\n\n %s %s %s\r\nA %06d REPT EVNT CDM\r\n \"%c:NA,%s,NSA,AUTO:\\\"\\\"\"\r\n;",
conf_content.slot_u.tid, mcp_date, mcp_time,
atag, aid,
ctx->new_drv_mgr_sta);
sendto_all_client(sendbuf);
atag++;
if(atag >= 0xffff)
{
atag = 0;
}
}
return 1;
}
void ext_bpp_evt(int aid, char bid)
{
unsigned char tmp[1024];
memset(tmp, '\0', 1024);
get_time_fromnet();
sprintf((char *)tmp, "%c%c%c%c%c%c%s%c", CR, LF, LF, SP, SP, SP, conf_content.slot_u.tid, SP);
sprintf((char *)tmp, "%s%s%c%s%c%c", tmp, mcp_date, SP, mcp_time, CR, LF);
sprintf((char *)tmp, "%sA%c%c%06d%cREPT%cEVNT%cC91%c%c", tmp, SP, SP, atag, SP, SP, SP, CR, LF);
sprintf((char *)tmp, "%s%c%c%c\"%c:NA,%c,NSA,AUTO:\\\"\\\"\"%c%c;",
tmp, SP, SP, SP, aid, bid, CR, LF);
sendto_all_client(tmp);
//printf("Send C91 %s\n",tmp);
atag++;
if(atag >= 0xffff)
{
atag = 0;
}
}
/*
1 成功
0 失败
*/
int ext_bid_cmp(u8_t ext_sid, u8_t *ext_new_data, struct extctx *ctx)
{
u8_t ext_old_bid[16];
u32_t tmp;
u8_t bppa[8];
int i;
memset(ext_old_bid, 0, 16);
if(EXT_1_MGR_PRI == ext_sid)
{
tmp = 0;
memcpy(ext_old_bid, ctx->extBid[0], 14);
if(0 != memcmp(ext_old_bid, ext_new_data, 14))
{
for(i = 0; i < 14; i++)
{
if(ext_old_bid[i] != ext_new_data[i])
{
if(EXT_NONE == ext_old_bid[i] && EXT_NONE != ext_new_data[i])
{
tmp &= (~BIT(i));
ext_alm_evt('1', 'k', 13 + i, "A ", "CL");
}
else if(EXT_NONE != ext_old_bid[i] && EXT_NONE == ext_new_data[i])
{
tmp |= BIT(i);
ext_alm_evt('1', 'k', 13 + i, "**", "MJ");
}
else
{
//do nothing
}
if(i < 10)
{
ext_bpp_evt(23 + i, ext_new_data[i]);
}
else if(10 == i)
{
ext_bpp_evt(EXT_1_MGR_PRI, ext_new_data[i]);
}
else if(11 == i)
{
ext_bpp_evt(EXT_1_MGR_RSV, ext_new_data[i]);
}
else if(12 == i)
{
ext_bpp_evt(EXT_1_PWR1, ext_new_data[i]);
}
else if(13 == i)
{
ext_bpp_evt(EXT_1_PWR2, ext_new_data[i]);
}
else
{
//do nothing
}
}
}
memset(bppa, 0, 8);
bppa[0] = 0x40 | (tmp & 0x0000000F);
bppa[1] = 0x40 | ((tmp >> 4) & 0x0000000F);
bppa[2] = 0x40 | ((tmp >> 8) & 0x0000000F);
bppa[3] = 0x40 | ((tmp >> 12) & 0x0000000F);
memcpy(&ctx->mgr[0].mgrAlm[3], bppa, 4);
}
}
else if(EXT_1_MGR_RSV == ext_sid)
{
tmp = 0;
memcpy(ext_old_bid, ctx->extBid[0], 14);
if(0 != memcmp(ext_old_bid, ext_new_data, 14))
{
for(i = 0; i < 14; i++)
{
if(ext_old_bid[i] != ext_new_data[i])
{
if(EXT_NONE == ext_old_bid[i] && EXT_NONE != ext_new_data[i])
{
tmp &= (~BIT(i));
ext_alm_evt('1', 'l', 13 + i, "A ", "CL");
}
else if(EXT_NONE != ext_old_bid[i] && EXT_NONE == ext_new_data[i])
{
tmp |= BIT(i);
ext_alm_evt('1', 'l', 13 + i, "**", "MJ");
}
else
{
//do nothing
}
if(i < 10)
{
ext_bpp_evt(23 + i, ext_new_data[i]);
}
else if(10 == i)
{
ext_bpp_evt(EXT_1_MGR_PRI, ext_new_data[i]);
}
else if(11 == i)
{
ext_bpp_evt(EXT_1_MGR_RSV, ext_new_data[i]);
}
else if(12 == i)
{
ext_bpp_evt(EXT_1_PWR1, ext_new_data[i]);
}
else if(13 == i)
{
ext_bpp_evt(EXT_1_PWR2, ext_new_data[i]);
}
else
{
//do nothing
}
}
}
memset(bppa, 0, 8);
bppa[0] = 0x40 | (tmp & 0x0000000F);
bppa[1] = 0x40 | ((tmp >> 4) & 0x0000000F);
bppa[2] = 0x40 | ((tmp >> 8) & 0x0000000F);
bppa[3] = 0x40 | ((tmp >> 12) & 0x0000000F);
memcpy(&ctx->mgr[1].mgrAlm[3], bppa, 4);
}
}
else if(EXT_2_MGR_PRI == ext_sid)
{
tmp = 0;
memcpy(ext_old_bid, ctx->extBid[1], 14);
if(0 != memcmp(ext_old_bid, ext_new_data, 14))
{
for(i = 0; i < 14; i++)
{
if(ext_old_bid[i] != ext_new_data[i])
{
if(EXT_NONE == ext_old_bid[i] && EXT_NONE != ext_new_data[i])
{
tmp &= (~BIT(i));
ext_alm_evt('2', 'k', 13 + i, "A ", "CL");
}
else if(EXT_NONE != ext_old_bid[i] && EXT_NONE == ext_new_data[i])
{
tmp |= BIT(i);
ext_alm_evt('2', 'k', 13 + i, "**", "MJ");
}
else
{
//do nothing
}
if(i < 10)
{
ext_bpp_evt(39 + i, ext_new_data[i]);
}
else if(10 == i)
{
ext_bpp_evt(EXT_2_MGR_PRI, ext_new_data[i]);
}
else if(11 == i)
{
ext_bpp_evt(EXT_2_MGR_RSV, ext_new_data[i]);
}
else if(12 == i)
{
ext_bpp_evt(EXT_2_PWR1, ext_new_data[i]);
}
else if(13 == i)
{
ext_bpp_evt(EXT_2_PWR2, ext_new_data[i]);
}
else
{
//do nothing
}
}
}
memset(bppa, 0, 8);
bppa[0] = 0x40 | (tmp & 0x0000000F);
bppa[1] = 0x40 | ((tmp >> 4) & 0x0000000F);
bppa[2] = 0x40 | ((tmp >> 8) & 0x0000000F);
bppa[3] = 0x40 | ((tmp >> 12) & 0x0000000F);
memcpy(&ctx->mgr[2].mgrAlm[3], bppa, 4);
}
}
else if(EXT_2_MGR_RSV == ext_sid)
{
tmp = 0;
memcpy(ext_old_bid, ctx->extBid[1], 14);
if(0 != memcmp(ext_old_bid, ext_new_data, 14))
{
for(i = 0; i < 14; i++)
{
if(ext_old_bid[i] != ext_new_data[i])
{
if(EXT_NONE == ext_old_bid[i] && EXT_NONE != ext_new_data[i])
{
tmp &= (~BIT(i));
ext_alm_evt('2', 'l', 13 + i, "A ", "CL");
}
else if(EXT_NONE != ext_old_bid[i] && EXT_NONE == ext_new_data[i])
{
tmp |= BIT(i);
ext_alm_evt('2', 'l', 13 + i, "**", "MJ");
}
else
{
//do nothing
}
if(i < 10)
{
ext_bpp_evt(39 + i, ext_new_data[i]);
}
else if(10 == i)
{
ext_bpp_evt(EXT_2_MGR_PRI, ext_new_data[i]);
}
else if(11 == i)
{
ext_bpp_evt(EXT_2_MGR_RSV, ext_new_data[i]);
}
else if(12 == i)
{
ext_bpp_evt(EXT_2_PWR1, ext_new_data[i]);
}
else if(13 == i)
{
ext_bpp_evt(EXT_2_PWR2, ext_new_data[i]);
}
else
{
//do nothing
}
}
}
memset(bppa, 0, 8);
bppa[0] = 0x40 | (tmp & 0x0000000F);
bppa[1] = 0x40 | ((tmp >> 4) & 0x0000000F);
bppa[2] = 0x40 | ((tmp >> 8) & 0x0000000F);
bppa[3] = 0x40 | ((tmp >> 12) & 0x0000000F);
memcpy(&ctx->mgr[3].mgrAlm[3], bppa, 4);
}
}
else if(EXT_3_MGR_PRI == ext_sid)
{
tmp = 0;
memcpy(ext_old_bid, ctx->extBid[2], 14);
if(0 != memcmp(ext_old_bid, ext_new_data, 14))
{
for(i = 0; i < 14; i++)
{
if(ext_old_bid[i] != ext_new_data[i])
{
if(EXT_NONE == ext_old_bid[i] && EXT_NONE != ext_new_data[i])
{
tmp &= (~BIT(i));
ext_alm_evt('3', 'k', 13 + i, "A ", "CL");
}
else if(EXT_NONE != ext_old_bid[i] && EXT_NONE == ext_new_data[i])
{
tmp |= BIT(i);
ext_alm_evt('3', 'k', 13 + i, "**", "MJ");
}
else
{
//do nothing
}
if(i < 3)
{
ext_bpp_evt(55 + i, ext_new_data[i]);
}
else if(i >= 3 && i < 10)
{
ext_bpp_evt(57 + i, ext_new_data[i]);
}
else if(10 == i)
{
ext_bpp_evt(EXT_3_MGR_PRI, ext_new_data[i]);
}
else if(11 == i)
{
ext_bpp_evt(EXT_3_MGR_RSV, ext_new_data[i]);
}
else if(12 == i)
{
ext_bpp_evt(EXT_3_PWR1, ext_new_data[i]);
}
else if(13 == i)
{
ext_bpp_evt(EXT_3_PWR2, ext_new_data[i]);
}
else
{
//do nothing
}
}
}
memset(bppa, 0, 8);
bppa[0] = 0x40 | (tmp & 0x0000000F);
bppa[1] = 0x40 | ((tmp >> 4) & 0x0000000F);
bppa[2] = 0x40 | ((tmp >> 8) & 0x0000000F);
bppa[3] = 0x40 | ((tmp >> 12) & 0x0000000F);
memcpy(&ctx->mgr[4].mgrAlm[3], bppa, 4);
}
}
else if(EXT_3_MGR_RSV == ext_sid)
{
tmp = 0;
memcpy(ext_old_bid, ctx->extBid[2], 14);
if(0 != memcmp(ext_old_bid, ext_new_data, 14))
{
for(i = 0; i < 14; i++)
{
if(ext_old_bid[i] != ext_new_data[i])
{
if(EXT_NONE == ext_old_bid[i] && EXT_NONE != ext_new_data[i])
{
tmp &= (~BIT(i));
ext_alm_evt('3', 'l', 13 + i, "A ", "CL");
}
else if(EXT_NONE != ext_old_bid[i] && EXT_NONE == ext_new_data[i])
{
tmp |= BIT(i);
ext_alm_evt('3', 'l', 13 + i, "**", "MJ");
}
else
{
//do nothing
}
if(i < 3)
{
ext_bpp_evt(55 + i, ext_new_data[i]);
}
else if(i >= 3 && i < 10)
{
ext_bpp_evt(57 + i, ext_new_data[i]);
}
else if(10 == i)
{
ext_bpp_evt(EXT_3_MGR_PRI, ext_new_data[i]);
}
else if(11 == i)
{
ext_bpp_evt(EXT_3_MGR_RSV, ext_new_data[i]);
}
else if(12 == i)
{
ext_bpp_evt(EXT_3_PWR1, ext_new_data[i]);
}
else if(13 == i)
{
ext_bpp_evt(EXT_3_PWR2, ext_new_data[i]);
}
else
{
//do nothing
}
}
}
memset(bppa, 0, 8);
bppa[0] = 0x40 | (tmp & 0x0000000F);
bppa[1] = 0x40 | ((tmp >> 4) & 0x0000000F);
bppa[2] = 0x40 | ((tmp >> 8) & 0x0000000F);
bppa[3] = 0x40 | ((tmp >> 12) & 0x0000000F);
memcpy(&ctx->mgr[5].mgrAlm[3], bppa, 4);
}
}
else
{
return 0;
}
return 1;
}
void ext_ssm_cfg(char *pSsm)
{
int i;
u8_t tmp_ctag[7];
u8_t sendbuf[256];
u8_t slotid;
memcpy(tmp_ctag, "000000", 6);
if( ((EXT_DRV == slot_type[12]) || (EXT_DRV == slot_type[13])) &&
((1 == gExtCtx.drv[0].drvPR[0]) || (1 == gExtCtx.drv[1].drvPR[0])) )
{
for(i = 0; i < 10; i++)
{
if((EXT_OUT16 == gExtCtx.extBid[0][i]) || (EXT_OUT32 == gExtCtx.extBid[0][i]) || (EXT_OUT16S == gExtCtx.extBid[0][i]) || (EXT_OUT32S == gExtCtx.extBid[0][i]))
{
// lev
memset(sendbuf, 0, 256);
sprintf((char *) sendbuf, "SET-OUT-LEV:%c:%s::%s;",
i + EXT_1_OUT_LOWER,
tmp_ctag,
pSsm );
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, i + EXT_1_OUT_LOWER, tmp_ctag);
}
}
}
if( ((EXT_DRV == slot_type[12]) || (EXT_DRV == slot_type[13])) &&
((1 == gExtCtx.drv[0].drvPR[1]) || (1 == gExtCtx.drv[1].drvPR[1])) )
{
for(i = 0; i < 10; i++)
{
if((EXT_OUT16 == gExtCtx.extBid[1][i]) || (EXT_OUT32 == gExtCtx.extBid[1][i]) || (EXT_OUT16S == gExtCtx.extBid[1][i]) || (EXT_OUT32S == gExtCtx.extBid[1][i]))
{
//lev
memset(sendbuf, 0, 256);
sprintf((char *) sendbuf, "SET-OUT-LEV:%c:%s::%s;",
i + EXT_2_OUT_LOWER,
tmp_ctag,
pSsm );
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, i + EXT_2_OUT_LOWER, tmp_ctag);
}
}
}
if( ((EXT_DRV == slot_type[12]) || (EXT_DRV == slot_type[13])) &&
((1 == gExtCtx.drv[0].drvPR[2]) || (1 == gExtCtx.drv[1].drvPR[2])) )
{
for(i = 0; i < 10; i++)
{
if((EXT_OUT16 == gExtCtx.extBid[2][i]) || (EXT_OUT32 == gExtCtx.extBid[2][i]) || (EXT_OUT16S == gExtCtx.extBid[2][i]) || (EXT_OUT32S == gExtCtx.extBid[2][i]))
{
if((i + EXT_3_OUT_LOWER) >= ':')//except ':;'
{
slotid = i + EXT_3_OUT_LOWER + 2;
}
else
{
slotid = i + EXT_3_OUT_LOWER;
}
//lev
memset(sendbuf, 0, 256);
sprintf((char *) sendbuf, "SET-OUT-LEV:%c:%s::%s;",
slotid,
tmp_ctag,
pSsm );
ext_issue_cmd(sendbuf, strlen_r(sendbuf, ';') + 1, slotid, tmp_ctag);
}
}
}
if((EXT_DRV == slot_type[12]) || (EXT_DRV == slot_type[13]))
{
for(i = 0; i < 30; i++)
{
memcpy(gExtCtx.save.outSsm[i], pSsm, 2);
memcpy(gExtCtx.out[i].outSsm, pSsm, 2);
}
ext_write_flash(&(gExtCtx.save));
}
}
int is_freq_input(unsigned char slot)
{
int i;
unsigned char port[2];
if(conf_content.slot_u.fb == '0')
{
if(slot == RB1_SLOT)
{
for(i = 0; i < 4; i++)
{
if(flag_alm[14][i + 4] == 0x00)
{
return 0;
}
}
}
else if(slot == RB2_SLOT)
{
for(i = 0; i < 4; i++)
{
if(flag_alm[15][i + 4] == 0x00)
{
return 0;
}
}
}
}
else
{
#if 1
if(slot_type[0] == 'S')
{
memset(port, 0, 2);
port[0] = alm_sta[0][5] & 0x0f;
port[1] = alm_sta[0][6] & 0x0f;
for(i = 9; i < 11; i++) //0x04
{
if( (i % 2) != 0 )
{
port[0] |= (alm_sta[0][i] & 0x0f);
}
else
{
port[1] |= (alm_sta[0][i] & 0x0f);
}
}
for(i = 5; i < 11; i++) //0x05
{
if( (i % 2) != 0 )
{
port[0] |= (alm_sta[18][i] & 0x0f);
}
else
{
port[1] |= (alm_sta[18][i] & 0x0f);
}
}
for(i = 0; i < 4; i++)
{
if( ((port[0] >> i) & 0x01) == 0x00)
{
return 0;
}
if( ((port[1] >> i) & 0x01) == 0x0)
{
return 0;
}
}
}
else
{
//nothing
}
if(slot_type[1] == 'S')
{
memset(port, 0, 2);
port[0] = alm_sta[1][5] & 0x0f;
port[1] = alm_sta[1][6] & 0x0f;
for(i = 9; i < 11; i++) //0x04
{
if( (i % 2) != 0 )
{
port[0] |= (alm_sta[1][i] & 0x0f);
}
else
{
port[1] |= (alm_sta[1][i] & 0x0f);
}
}
for(i = 5; i < 11; i++) //0x05
{
if( (i % 2) != 0 )
{
port[0] |= (alm_sta[19][i] & 0x0f);
}
else
{
port[1] |= (alm_sta[19][i] & 0x0f);
}
}
for(i = 0; i < 4; i++)
{
if( ((port[0] >> i) & 0x01) == 0x00)
{
return 0;
}
if( ((port[1] >> i) & 0x01) == 0x00)
{
return 0;
}
}
}
#endif
}
return 1;
}
//检查是否为2级时钟,小于等于G.812
int timeClock_2(const unsigned char rb)
{
unsigned char port;
unsigned char slot = 0;
if('0' == conf_content.slot_u.fb)
{
switch(rpt_content.slot_o.rb_ref)
{
case '5':
return 0;
break;
case '6':
return 0;
break;
case '7':
return 0;
break;
case '8':
return 0;
break;
default:
return 0;
break;
}
}
else
{
//后面板:3--reftf1 时间;4--reftf2时间;5--reftf1频率;6--reftf2频率
if('o' == rb)//计算reftf槽位号
{
if('3' == rpt_content.slot_o.rb_ref || '4' == rpt_content.slot_o.rb_ref)//后面板时间输入
{
slot = rpt_content.slot_o.rb_ref - '3';
}
else if('5' == rpt_content.slot_o.rb_ref || '6' == rpt_content.slot_o.rb_ref)//后面板频率输入
{
slot = rpt_content.slot_o.rb_ref - '5';
}
else
{
printf("RB up pps_stat error\n");
}
}
else
{
if('3' == rpt_content.slot_p.rb_ref || '4' == rpt_content.slot_p.rb_ref)//后面板时间输入
{
slot = rpt_content.slot_p.rb_ref - '3';
}
else if('5' == rpt_content.slot_p.rb_ref || '6' == rpt_content.slot_p.rb_ref)//后面板频率输入
{
slot = rpt_content.slot_p.rb_ref - '5';
}
else
{
printf("RB up pps_stat error\n");
}
}
//确认端口号
if('3' == rpt_content.slot_o.rb_ref || '4' == rpt_content.slot_o.rb_ref)
{
if( rpt_content.slot[slot].ref_mod2[2] == '\0' || rpt_content.slot[slot].ref_mod2[2] == '0')
{
return 0;
}
else
{
port = rpt_content.slot[slot].ref_mod2[2] - '1';
}
}
else
{
if( rpt_content.slot[slot].ref_mod1[2] == '\0' || rpt_content.slot[slot].ref_mod1[2] == '0')
{
return 0;
}
else
{
port = rpt_content.slot[slot].ref_mod1[2] - '1';
}
}
//检查时钟质量等级
if( rpt_content.slot[slot].ref_tl[port] != 'a')
{
return 1;
}
else
{
return 0;
}
}
}
int get_tod_sta(unsigned char *buf, unsigned char slot)
{
//int flag_a;
unsigned char res;
if(slot == RB1_SLOT)
{
if(strncmp1(rpt_content.slot_o.rb_msmode, "MAIN", 4) == 0)
{
//flag_a=is_freq_input('o');
if(buf[0] == '1')
{
if ( rpt_content.slot_o.rb_ref == '3' ||
rpt_content.slot_o.rb_ref == '4' ||
rpt_content.slot_o.rb_ref == '5' ||
rpt_content.slot_o.rb_ref == '6' ||
rpt_content.slot_o.rb_ref == '7' ||
rpt_content.slot_o.rb_ref == '8' )
{
res = timeClock_2('o');
if( res && ( '1' == conf_content3.mcp_protocol) )
{
buf[0] = '5';//二级时钟
}
else
{
buf[0] = '1'; //正常跟踪一级时钟
}
}
else//正常跟踪卫星
{
buf[0] = '0';
}
}
else if(buf[0] == '2')
{
buf[0] = '5'; //保持
}
else if(buf[0] == '3')
{
//不可用
if( '1' == conf_content3.mcp_protocol )
{
buf[0] = '7';
}
else
{
buf[0] = '2';
}
}
else if(buf[0] == '4')
{
//XO保持
if( '1' == conf_content3.mcp_protocol )
{
buf[0] = '4';
}
else
{
buf[0] = '3';
}
}
else
{
return 1;
}
}
else
{
return 1;
}
}
else if(slot == RB2_SLOT)
{
if(strncmp1(rpt_content.slot_p.rb_msmode, "MAIN", 4) == 0)
{
//flag_a=is_freq_input('o');
if(buf[0] == '1')
{
if ( rpt_content.slot_p.rb_ref == '3' ||
rpt_content.slot_p.rb_ref == '4' ||
rpt_content.slot_p.rb_ref == '5' ||
rpt_content.slot_p.rb_ref == '6' ||
rpt_content.slot_p.rb_ref == '7' ||
rpt_content.slot_p.rb_ref == '8' )
{
res = timeClock_2('p');
if( res && ( '1' == conf_content3.mcp_protocol) )
{
buf[0] = '5';//二级时钟
}
else
{
buf[0] = '1'; //正常跟踪一级时钟
}
}
else//正常跟踪卫星
{
buf[0] = '0';
}
}
else if(buf[0] == '2')
{
buf[0] = '5'; //保持
}
else if(buf[0] == '3')
{
//不可用
if( '1' == conf_content3.mcp_protocol )
{
buf[0] = '7';
}
else
{
buf[0] = '2';
}
}
else if(buf[0] == '4')
{
//XO保持
if( '1' == conf_content3.mcp_protocol )
{
buf[0] = '4';
}
else
{
buf[0] = '3';
}
}
else
{
return 1;
}
}
else
{
return 1;
}
}
else
{
return 1;
}
return 0;
}
int send_tod(unsigned char *buf, unsigned char slot)
{
unsigned char sendbuf1[SENDBUFSIZE];
unsigned char sendbuf2[SENDBUFSIZE];
unsigned char *ctag = "000000";
int i;
memset(sendbuf1, '\0', SENDBUFSIZE);
memset(sendbuf2, '\0', SENDBUFSIZE);
if(get_tod_sta(buf, slot) == 1)
{
return 1;
}
//modified by zhanghui 2013-9-10 15:50:12
if('2' == buf[2])
{
buf[2] = '3';
}
else if(('3' == buf[2]))
{
buf[2] = '2';
}
else
{
//do nothing
}
//buf[0]=TOD_STA;
sprintf((char *)sendbuf1, "SET-TOD-STA:%s::0,%s;", ctag, buf);
sprintf((char *)sendbuf2, "SET-PTP-STA:%s::%s;", ctag, buf);
for(i = 0; i < 14; i++)
{
if(slot_type[i] == 'I')
{
sendtodown(sendbuf1, (unsigned char)(i + 0x61));
printf("+++++tod:%s\n",sendbuf1);
}
else if(((slot_type[i] < 'I') && (slot_type[i] > '@')) || (slot_type[i] == 'j') )
{
sendtodown(sendbuf2, (unsigned char)(i + 0x61));
printf("-----tod:%s\n",sendbuf2);
}
}
return 0;
}
void rb_do_nothing(void)
{
}
void rb_16_master(void)
{
sendtodown("SET-MAIN:000000::MAIN;",'p');
printf("RB auto master\n");
}
void rb_16_salve(void)
{
sendtodown("SET-MAIN:000000::STBY;",'p');
printf("RB auto STBY\n");
}
void lct_set_rb(void)
{
if(strncmp(conf_content.slot_p.msmode,"STBY",4) == 0)
{
sendtodown("SET-MAIN:000000::STBY;",'p');
}
else
{
sendtodown("SET-MAIN:000000::MAIN;",'p');
}
printf("RB LCT\n");
}
int rb_stat_index(int slot)
{
unsigned char stat_buf[5];
memset(stat_buf,0,5);
if(slot == 14)
{
if('O' == slot_type[14])
{
return 0;
}
else
{
memcpy(stat_buf,rpt_content.slot_o.rb_trmode,4);
}
}
if(slot == 15)
{
if('O' == slot_type[15])
{
return 0;
}
else
{
memcpy(stat_buf,rpt_content.slot_p.rb_trmode,4);
}
}
if(stat_buf == NULL)
return -1;
if( strncmp(stat_buf,"FREE",4)==0 )
{
return 1;
}
else if( strncmp(stat_buf,"FATR",4)==0 )
{
return 2;
}
else if( strncmp(stat_buf,"TRCK",4)==0 )
{
return 3;
}
else if( strncmp(stat_buf,"HOLD",4)==0 )
{
return 4;
}
else
{
return -1;
}
}
/*
16#RB pan state
-------------------------------------------------------------------------------
| \ 16#| |
|15# \ | None FreeRun FastTrack Locked HoldOver |
|------------------------------------------------------------------------------|
|None | None Master Master Master Master |
| | |
|FreeRun | None Master Master Master Master |
| | |
|FastTrack| None slave LCT Master Master |
| | |
|Locked | None slave slave LCT slave |
| | |
|HoldOver | None slave slave Master LCT |
-------------------------------------------------------------------------------
*/
typedef void (*Func_Matrix)(void);
void timer_rb_ms(void)
{
signed char i,j;
static signed char rb_stat_index_save = 0;
Func_Matrix rb_ms_change[5][5] = {
{rb_do_nothing, rb_16_master, rb_16_master, rb_16_master, rb_16_master},
{rb_do_nothing, rb_16_master, rb_16_master, rb_16_master, rb_16_master},
{rb_do_nothing, rb_16_salve , lct_set_rb , rb_16_master, rb_16_master},
{rb_do_nothing, rb_16_salve , rb_16_salve , lct_set_rb , rb_16_salve },
{rb_do_nothing, rb_16_salve , rb_16_salve , rb_16_master, lct_set_rb }
};
i = rb_stat_index(14);
j = rb_stat_index(15);
if(i==-1 || j ==-1)
{
return ;
}
if( rb_stat_index_save == i*5+j )
{
return ;
}
else
{
rb_stat_index_save = i*5+j;
}
rb_ms_change[i][j]();
}
/*
mcp定时检测控制
*/
void timer_mcp(void * loop)
{
bool_t *loop_flag = loop;
while(!(*loop_flag))
{
sleep(120);
timer_rb_ms();
}
printf("The RB MasterSlave pthread exit!\n");
pthread_exit(NULL);
}
<file_sep>/MCPU/mcp_save_struct.h
#ifndef _MCP_SAVE_STRUCT_H_
#define _MCP_SAVE_STRUCT_H_
#pragma pack(1)
typedef struct
{
unsigned char sy_ver[40];
unsigned char tp16_en[17];
unsigned char tp16_tod[4];
unsigned char out_lev[3];
unsigned char out_typ[17];
unsigned char out_sta;
unsigned char out_mode;
unsigned char ptp_ip[4][16];
unsigned char ptp_mac[4][18];
unsigned char ptp_gate[4][16];
unsigned char ptp_mask[4][16];
unsigned char ptp_dns1[4][16];
unsigned char ptp_dns2[4][16];
/*<eable>,<delaytype>,<multicast>,<enp>,<step>,
<sync>,<announce>,<delayreq>,<pdelayreq>,
<delaycom>,<linkmode>
delete the port*/
unsigned char ptp_en[4];
unsigned char ptp_delaytype[4];
unsigned char ptp_multicast[4];
unsigned char ptp_enp[4];
unsigned char ptp_step[4];
unsigned char ptp_sync[4][3];
unsigned char ptp_announce[4][3];
unsigned char ptp_delayreq[4][3];
unsigned char ptp_pdelayreq[4][3];
unsigned char ptp_delaycom[4][9];
unsigned char ptp_linkmode[4];
unsigned char ptp_out[4];
unsigned char ptp_clkcls[4][4];
//unsigned char ptp_dom[4];
unsigned char ref_prio1[9];
unsigned char ref_prio2[9];
unsigned char ref_mod1[4];
unsigned char ref_mod2[4];
unsigned char ref_ph[128];
unsigned char ref_sa[9];
unsigned char ref_typ1[9];
unsigned char ref_typ2[9];
unsigned char ref_tl[9];
unsigned char ref_en[9];
unsigned char ref_ssm_en[9];
unsigned char rs_en[5];
unsigned char rs_tzo[5];
unsigned char ppx_mod[17];
unsigned char tod_en[17];
unsigned char tod_br;
unsigned char tod_tzo;
unsigned char igb_en[5];
unsigned char igb_rat[5];
unsigned char igb_max[5];
unsigned char igb_tzone;
unsigned char ntp_ip[9];
unsigned char ntp_mac[13];
unsigned char ntp_gate[9];
unsigned char ntp_msk[9];
unsigned char ntp_bcast_en;
unsigned char ntp_tpv_en;
unsigned char ntp_md5_en;
unsigned char ntp_ois_en;
unsigned char ntp_interval[5];
unsigned char ntp_key_flag[4];
unsigned char ntp_keyid[4][5];
unsigned char ntp_key[4][17];
unsigned char ntp_ms[26];
unsigned char ntp_leap[4];
unsigned char ntp_mcu[2];
unsigned char ntp_pmac[12][6];
unsigned char ntp_pip[12][5];
unsigned char ntp_pnetmask[12][4];
unsigned char ntp_pgateway[12][4];
unsigned char ntp_portEn[5];
unsigned char ntp_portStatus[5];
unsigned char ref_2mb_lvlm;
} rpt_out_content;
typedef struct
{
/* <responsemessage>::=“<aid>,<type>,[<version-MCU>],[<version-FPGA>],
[<version-CPLD>],[<version-PCB>],<ref>,<priority>,<mask>,<leapmask>,<leap>,<leaptag>,
[<delay>],[<delay>],[<delay>],[<delay>],[<delay>],[<delay>],[<delay>],[<delay>],
<we>,<out>,<type-work>,<msmode>,<trmode>”*/
unsigned char rb_ref;
unsigned char rb_prio[9];
unsigned char rb_mask[9];
unsigned char rb_leapmask[6];
unsigned char rb_leap[3];
unsigned char rb_leaptag;
unsigned char rf_dey[8][9];/*<deley>X8*/
unsigned char rf_tzo[4];/*<we>,<out>*/
unsigned char rb_typework[8];
unsigned char rb_msmode[5];
unsigned char rb_trmode[5];
unsigned char sy_ver[40];
unsigned char rb_inp[10][128];
unsigned char rb_tl;
unsigned char rb_sa;
unsigned char rb_tl_2mhz;
unsigned char tod_buf[4];
unsigned char rb_phase[2][9];
unsigned char rb_ph[7][10];// #17 #18 #1 time #2 time #1 fre #2 fre
} rpt_rb_content;
typedef struct
{
/*<response message >::= “
<aid>,<type>,[<version-MCU>],[<version-FPGA>], [<version-CPLD>],[<version-PCB>],
<ppssta>,<sourcetype>,<pos_mode>,<elevation>, <format>, <acmode>, <mask>,
<qq-fix>,<hhmmss.ss>,<ddmm.mmmmmm>,<ns>,<dddmm.mmmmmm>,<we>,<saaaaa.aa>,
<vvvv>,<tttttttttt>[,<sat>,<qq>,<pp>,<ss>,<h>]*”*/
unsigned char sy_ver[40];
unsigned char gb_sta;
unsigned char gb_tod[4];
unsigned char gb_pos_mode[5];
unsigned char gb_elevation[9];
unsigned char gb_format[6];
unsigned char gb_acmode[4];
unsigned char gb_mask[3];/*<mask>*/
unsigned char gb_ori[70];/*<qq>,<hhmmss.ss>,<ddmm.mmmmmm>,<ns>,<dddmm.mmmmmm>,<we>,<saaaaa.aa>,
<vvvv>,<tttttttttt>*/
//unsigned char gb_rev[256];
unsigned char gb_rev_g[256]; /*<sat>,<qq>[,<pp>,<ss>,<h>]**/
unsigned char gb_rev_b[256];
unsigned char gb_rev_glo[256]; /*<sat>,<qq>[,<pp>,<ss>,<h>]**/
unsigned char gb_rev_gal[256];
unsigned char bdzb[8];
unsigned char staen;
unsigned char rec_type[12];
unsigned char rec_ver[40];
unsigned char leapnum[4];
unsigned char leapalm[3];
unsigned char delay[8];
unsigned char pps_out_delay[16];
} rpt_gbtp_content;
typedef struct
{
rpt_out_content slot[14];
rpt_rb_content slot_o, slot_p;
rpt_gbtp_content slot_q, slot_r;
} rpt_all_content;
typedef struct
{
unsigned char ptp_prio1[14][4][5];//14个槽位,4个端口,5个字节保存信息。
unsigned char ptp_prio2[14][4][5];
unsigned char ptp_mtc_ip[14][4][152];//保存单播ip
unsigned char ptp_dom[14][4][4];//20170616 add
unsigned char esmc_en[14][4];
unsigned char ptp_ver[14][4][10];
unsigned char sfp_type[14][4][3];
unsigned char sfp_oplo[14][4][7];
unsigned char sfp_ophi[14][4][7];
unsigned char time_source[14][4][5];
unsigned char clock_class[14][4][4];
unsigned char time_acc[14][4][5];
unsigned char synce_ssm[14][4][14];
} file_ptp_mtc; //第一部分配置信息由file_content保存,第二部分为扩展框,此为第三部分。
#pragma pack()
rpt_all_content rpt_content;
file_ptp_mtc rpt_ptp_mtc;
//
//define esmc enable,ptp_domain.
//
//unsigned char esmc_en[14][4];
//unsigned char ptp_dom[14][4][4];
void sta1_alm(unsigned char *sendbuf, unsigned char slot);
void sta1_portnum(unsigned char *sendbuf, unsigned char slot);
void sta1_net(unsigned char *sendbuf, unsigned char slot);
void sta1_cpu(unsigned char *sendbuf, unsigned char slot);
void sta1_in_out(unsigned char *sendbuf, unsigned char slot);
void set_sta_ip(unsigned const char *ip, unsigned char solt);
void set_sta_gateway(unsigned char *ip, unsigned char solt);
void set_sta_netmask(unsigned char *ip, unsigned char solt);
//extern int _NUM_REVT;
#endif
<file_sep>/MCPU/mcp_main.c
#include <sys/resource.h>
#include <stdio.h>
#include <errno.h>
#include <signal.h>
//#include <linux/tcp.h>
#include <netinet/tcp.h>
#include "ext_alloc.h"
#include "ext_global.h"
#include "mcp_main.h"
#include "mcp_process.h"
#include "mcp_def.h"
#include "mcp_save_struct.h"
#include "mcp_set_struct.h"
//#include "memwatch.h"
//#include "memwatch.h"
int qid;
int print_switch = 0;
char TOD_STA = '2';
static bool_t Loop_Mark = false;
void log()
{
printf("****************************************************\n");
printf("* *\n");
printf("* *\n");
printf("* P300 TF EXT *\n");
printf("* *\n");
printf("*v%s.%s.%s.%s_%c \n",VER_MAJOR,VER_MINOR,VER_REVIS,VER_DATE,VER_STATE);
printf("* *\n");
printf("****************************************************\n");
}
void black_door(int sig)
{
printf("+++++signal is %d\n",sig);
TOD_STA = sig+'0';
}
int main(int argc , char **argv)
{
//
//!Socket Attribute Setting.
//
int opt = 1;
//
//!Bind The Local IP and Port Parameters.
//
struct sockaddr_in server;
//
//!Process pid Getting.
//
pid_t pid;
//
//! Priority Setting.
//
int prio;
int i, j, len_rpt;
ssize_t len;
int tmp, temp;
//
//! Tcp Receive Buffer and Data Swap Buffer.
unsigned char recvbuf[MAXRECVDATA];
unsigned char rpt_buf[MAXDATASIZE];
//const char chOpt=1;
//int nErr;
struct timeval timeout = {0, 100000};
//int flag_slotOIsMaster =0; //2014-1-27 rb备用板是否为主用状态;1启用备槽
printf("sizeof(file_content)=%d\n", (int)sizeof(file_content));
printf("sizeof(file_content3)=%d\n",(int)sizeof(file_content3));
//
//Get self-process pid.
//
pid = getpid();
//
//Set self-process priority.
//
if (setpriority(PRIO_PROCESS, pid, -12) != 0)
{
printf("%s%s\n", "MCPU", "Can't set ntp's priority!");
}
//
//Record The Last error.
errno = 0;
//
//!Get Self-Process Priority.
prio = getpriority(PRIO_PROCESS, pid);
if ( 0 == errno )
{
printf("MCPU priority is %d\n", prio);
}
FpgaWrite( WdControl, 0x00 );
FpgaWrite( WdControl, 0x01 );
log();
Init_filesystem();
initializeContext(&gExtCtx);
init_var();
config_enet();
Open_FpgaDev();
//
//Timer 120s Check The Setting or not Return Corrected.
init_timer();
qid = msgQ_create(PATH_NAME, PROJ_ID);
//
//! Create The Thread of deal RB Master/Slave inter 60 s.
//
if(Create_Thread(&Loop_Mark) == false)
{
printf("<MCPU ERR> %s\n", "Create RB Mastr Slave Thread Failed!");
return(-1);
}
#if 0
if(SIG_ERR == signal(1,black_door))
{
perror("++++++signal1\n");
}
if(SIG_ERR == signal(2,black_door))
{
perror("++++++signal2\n");
}
if(SIG_ERR == signal(3,black_door))
{
perror("++++++signal3\n");
}
if(SIG_ERR == signal(4,black_door))
{
perror("++++++signal4\n");
}
if(SIG_ERR == signal(5,black_door))
{
perror("++++++signal5\n");
}
if(SIG_ERR == signal(6,black_door))
{
perror("++++++signal6\n");
}
if(SIG_ERR == signal(7,black_door))
{
perror("++++++signal7\n");
}
#endif
#if DEBUG_NET_INFO
printf("%s:init completed,create socket...\n", NET_INFO);
#endif
if ((listenfd = socket(AF_INET, SOCK_STREAM, 0)) == -1)
{
perror("Creating socket failed.");
exit(1);
}
//允许多客户端连接
setsockopt(listenfd, SOL_SOCKET, SO_REUSEADDR, &opt, sizeof(opt)); //设置socket属性
setsockopt(listenfd, SOL_SOCKET, SO_SNDTIMEO, &timeout, sizeof(timeout));
setsockopt(listenfd, SOL_SOCKET, SO_RCVTIMEO, &timeout, sizeof(timeout));
// if(nErr==-1)
//{
// perror("TCP_NODELAY error\n");
//return ;
//}
bzero(&server, sizeof(server));
server.sin_family = AF_INET;
server.sin_port = htons(PORT);
// server.sin_addr.s_addr = inet_addr("192.168.1.10");
server.sin_addr.s_addr = htonl(INADDR_ANY);
if (bind(listenfd, (struct sockaddr *)&server, sizeof(struct sockaddr)) == -1)
{
//调用bind绑定地址
perror("Bind error.");
exit(1);
}
if (listen(listenfd, BACKLOG) == -1)
{
//调用listen开始监听
perror("listen() error\n");
exit(1);
}
#if DEBUG_NET_INFO
printf("%s:listening...\n", NET_INFO);
#endif
//
//!初始化select
//
maxfd = listenfd;
maxi = -1;
for(client_num = 0; client_num < MAX_CLIENT; client_num++)
{
client_fd[client_num] = -1;
}
//
//!
//
FD_ZERO(&allset); //清空
FD_SET(listenfd, &allset); //将监听socket加入select检测的描述符集合
print_switch = 1;
while (1)
{
struct sockaddr_in addr;
rset = allset;
// int select(int nfds, fd_set *readfds, fd_set *writefds,fd_set *exceptfds, struct timeval *timeout);
//! timeout == NULL:block the tcp connected.
nready = select(maxfd + 1, &rset, NULL, NULL, NULL); //调用select
//
//! select error,continue;
//if(nready <= 0)
//{
// perror("select error\n");
// continue;
//}
//else
if (FD_ISSET(listenfd, &rset)) //检测是否有新客户端请求
{
//fprintf(stderr,"f:Accept a connection.\n");
sin_size = sizeof(struct sockaddr_in);
if ((connectfd = accept(listenfd, (struct sockaddr *)&addr, (socklen_t *) & sin_size)) == -1)
{
continue;
}
//将新客户端的加入数组
for (client_num = 0; client_num < MAX_CLIENT; client_num++)
{
if (client_fd[client_num] < 0)
{
client_fd[client_num] = connectfd; //保存客户端描述符
//if(client_num == 0)
//{
//init_pan();
//}
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:Got a new Client<%d>\n", NET_INFO, client_num);
}
#endif
break;
}
}
if (client_num >= MAX_CLIENT)
{
printf("Too many clients\n");
close(connectfd);
}
else
{
FD_SET(connectfd, &allset);
if (connectfd > maxfd)
{
maxfd = connectfd; //确认maxfd是最大描述符
}
if (client_num > maxi) //数组最大元素值
{
maxi = client_num;
}
if (--nready <= 0)
{
continue;
}
}//如果没有新客户端连接,继续循环
}
for (client_num = 0; client_num <= maxi; client_num++)
{
if ((sockfd = client_fd[client_num]) < 0) //如果客户端描述符小于0,则没有客户端连接,检测下一个
{
continue;
}
// 有客户连接,检测是否有数据
if (FD_ISSET(sockfd, &rset))
{
memset(recvbuf, '\0', MAXRECVDATA);
//len = recv(sockfd, recvbuf, MAXDATASIZE, 0);
if ((len = recv(sockfd, recvbuf, MAXDATASIZE, 0)) <= 0)
{
close(sockfd);
FD_CLR(sockfd, &allset);
client_fd[client_num] = -1;
#if DEBUG_NET_INFO
if(print_switch == 0)
{
printf("%s:client<%d>closed. \n", NET_INFO, client_num);
}
#endif
}
// else if (len < 0)
// {
// }
else
{
#if 1
if(len < 8)
{
/*<OL:xxx;>*/
/*@@{ 00000000 00000000 00000000*/
/*OL:oo?;*/
//判断取盘信息
if(strncmp1("OL", recvbuf, 2) == 0)
{
for(i = 0; i < 3; i++)
{
for(j = 0; j < 8; j++)
{
tmp = ((recvbuf[3 + i] >> j) & 0x01);
if(tmp == 0x00) //not online
{
temp = i * 8 + j;
//printf("not online temp:%d\n ",temp);
if(temp < 0 || temp > 23)
{
printf("<not online>temp out of range\n");
}
if(online_sta[i * 8 + j] == 0x01)
{
//send not online
send_online_framing(temp, 'O');
/*取盘后,删除以前上报数据*/
if((temp) < 14)
{
memset(&rpt_content.slot[temp], '\0', sizeof(out_content));
}
else if(temp == 14)
{
memset(&rpt_content.slot_o, '\0', sizeof(rb_content));
//flag_slotOIsMaster = 0;
}
else if(temp == 15)
{
memset(&rpt_content.slot_p, '\0', sizeof(rb_content));
}
else if(temp == 16)
{
memset(&rpt_content.slot_q, '\0', sizeof(gbtp_content));
}
else if(temp == 17)
{
memset(&rpt_content.slot_r, '\0', sizeof(gbtp_content));
}
memset(alm_sta[temp], 0, 14);
send_pullout_alm(temp + 24, 0);
}
online_sta[temp] = tmp;
slot_type[temp] = 'O';
}
else //online
{
temp = i * 8 + j;
if(temp < 0 || temp > 23)
{
printf("<online>temp out of range\n");
}
if(online_sta[temp] == 0x00)
{
if(temp == 22)
{
send_online_framing(temp, 'Q');
slot_type[temp] = 'Q';
}
else if((temp == 18) || (temp == 19))
{
//send online
send_online_framing(temp, 'M');
slot_type[temp] = 'M';
//init_pan(temp);
}
//else if((temp==20)||(temp==21))
else if(temp == 20)
{
//send online
send_online_framing(temp, 'N');
slot_type[temp] = 'N';
init_pan(20);
}
else if((temp == 16) || (temp == 17))
{
//send online
//send_online_framing(temp,'L');
//slot_type[temp]='L';
}
else if((temp == 14) || (temp == 15))
{
//send online
// send_online_framing(temp,'K');
// slot_type[temp]='K';
//init_pan((char)(temp+'a'));
//if(temp==14)
//printf("online\n");
}
if(temp >= 0 && temp < 24)
{
send_pullout_alm(temp + 24, 1);
}
}
online_sta[temp] = tmp;
}
}
}
}
/*
if(0 == online_sta[15])//2014-1-27 rb主框出问题时启用备用框
{
if(0 == flag_slotOIsMaster && slot_type[14]!= 'O')
{
//printf("set-main:main\n");
sendtodown("SET-MAIN:000000::MAIN;",'o');
flag_slotOIsMaster = 1;
}
}
else
{
if(1 == flag_slotOIsMaster && slot_type[15]!= 'O')
{
flag_slotOIsMaster = 0;
sendtodown("SET-MAIN:000000::STBY;",'o');
}
}
*/
}
else
{
for(i = 0, j = 0; i < 1024; i++)
{
if(recvbuf[i] == ';')
{
len_rpt = i - j + 1;
memset(rpt_buf, '\0', sizeof(unsigned char) * MAXDATASIZE);
memcpy(rpt_buf, &recvbuf[j], len_rpt);
judge_data(rpt_buf, len_rpt, client_fd[client_num]);
j = i + 1;
}
}
}
#endif
}
if (--nready <= 0)
{
break;
}
}
}
#if 1
if(1 == reset_network_card)
{
if(0 == shake_hand_count)
{
//printf("phy reset\r\n");
mcpd_reset_network_card();
}
shake_hand_count = 0;
reset_network_card = 0;
}
#endif
}
close(listenfd); //关闭服务器监听socket
Close_FpgaDev();
return 0;
}
<file_sep>/MCPU/ext_cmn.h
#ifndef __EXT_CMN__
#define __EXT_CMN__
int strlen_r( char *str , char end);
#endif//__EXT_CMN__
<file_sep>/MCPU/mcp_def.h
#ifndef _MCP_DEF_H_
#define _MCP_DEF_H_
#define BaseAddr1 (0x03600000) //write
#define BaseAddr2 (0x03600000 | 0x04000000) //read
#define REG16_FPGA(BaseAddr, Addr) (BaseAddr + Addr)
#define ALMControl REG16_FPGA(BaseAddr1,0x0022)
#define FPGA_READ 0x01
#define FPGA_WRITE 0x02
#define WdControl REG16_FPGA(BaseAddr1,0x0020)
#define IndicateLED REG16_FPGA(BaseAddr1,0x0030)
#define TCP_NETWORK_CARD "eth0" //网卡
typedef unsigned char Uint8;
typedef unsigned short Uint16;
typedef unsigned long Ulong32;
typedef short int Int16;
typedef char Int8;
typedef long Long32;
typedef float Float32;
typedef double Double64;
unsigned char mcp_date[11];
unsigned char mcp_time[9];
unsigned char password[9];
unsigned short atag;
unsigned char slot_type[24];
unsigned char fpga_ver[7];
int online_sta[24];
//
//! ref pge4s alarm 0x50.
//
int flag_alm[36][26]; //20:ref1 21:ref2 22-35 PGE4S
unsigned char alm_sta[37][14];//18:ref1 19:ref2 23-36 PGE4S
int flag_i_o;
int flag_i_p;
int timeout_cnt[MAX_CLIENT];
unsigned char saved_alms[SAVE_ALM_NUM][50];
int saved_alms_cnt;
int time_temp;
fd_set rset, allset;
int client_fd[MAX_CLIENT];
extern int qid; /*消息队列标识符*/
int listenfd, maxfd, connectfd, sockfd;
int client_num, maxi, nready, count_data;
int sin_size; //地址信息结构体大小
int client_num;
unsigned char event_old[17][128];
int g_DrvLost;
int g_LeapFlag;
extern int _CMD_NODE_MAX;
extern int _NUM_RPT100S;
extern int NUM_REVT;
#endif
| e6f31ca91721a1efab2d6cf1b55c0e944a3f1292 | [
"C",
"Makefile"
] | 23 | C | isliulin/TimeFrequency | 707804e73618de356d1a815683de34ef20bcd0a8 | 72c3153c98607cf3861a4bb64dcccdc5a6c87384 | |
refs/heads/master | <repo_name>yeti-iasof/bx-integration<file_sep>/src/components/EmitedLabesList/EmitedLabesList.js
import React from 'react';
const EmitedLabesList = (props) => {
if (props.rows === undefined)
return null;
return (
<div className="table-container">
<table className="table">
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Nro Tracking</th>
<th scope="col">Cuenta Corriente</th>
<th scope="col">Fecha Creacion</th>
<th scope="col">Referencias</th>
</tr>
</thead>
<tbody>
{
props.rows.map((row, x) => {
return (
<tr key={x}>
<td scope="row">{x}</td>
<td scope="col">{row.nroOS}</td>
<td scope="col">{row.ctaCte}</td>
<td scope="col">{row.fechaCreacion}</td>
<td scope="col">{row.numeroReferencia}</td>
</tr>);
})
}
</tbody>
</table>
</div>
);
}
EmitedLabesList.propTypes = {};
EmitedLabesList.defaultProps = {};
export default EmitedLabesList;
<file_sep>/src/components/Home/Home.js
import React from 'react';
import styles from './Home.module.css';
import Header from '../Header/Header';
import Sider from '../Sider/Sider';
import ShowMenuSelected from '../ShowMenuSelected/ShowMenuSelected';
const Home = (props) => (
<div className={styles.Home}>
<Header onClickLogout={props.onClickLogout} username={props.username} />
<div className="d-flex">
<Sider menus={props.menus} onclickMenuOption={props.onclickMenuOption} />
<ShowMenuSelected currentMenu={props.currentMenu} baseUrl={props.baseUrl} bxToken={props.bxToken} bxUserCode={props.bxUserCode} bxClientAccout={props.bxClientAccout}/>
</div>
</div>
);
Home.propTypes = {};
Home.defaultProps = {};
export default Home;
<file_sep>/src/components/EmissionLaPolar/EmissionLaPolar.js
import React, { useState } from 'react';
import LockedWindow from '../LockedWindow/LockedWindow';
import * as XLSX from 'xlsx';
import './EmissionLaPolar.css';
import axios from 'axios';
import swal from 'sweetalert';
import * as EmailValidator from 'email-validator';
const ExcelDataLayout = (props) => {
if (props.excel === undefined)
return null;
return (
<div className="table-container">
{
props.excel.map((sheet, i) => {
return (
<table className="table" key={i}>
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">{sheet.sheetName}</th>
</tr>
</thead>
<tbody>
{
sheet.data.map((row, x) => {
return (
<tr key={x}>
<th scope="row">{x}</th>
<td>{JSON.stringify(row)}</td>
</tr>);
})
}
</tbody>
</table>
)
})
}
</div>
);
}
const EmissionLaPolar = (props) => {
const [filename, setFilename] = useState('Choose File');
const [loading, setLoading] = useState(false);
const [excel, setExcel] = useState();
const [emailNotification, setEmailNotification] = useState(undefined);
const handleFileChange = (event) => {
event.preventDefault();
if (event.target.files[0] === undefined)
return false;
if (event.target.files[0].type !== "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet")
return false;
setFilename(event.target.files[0].name);
setLoading(true);
const target = event.target
let hojas = [];
let reader = new FileReader()
reader.readAsArrayBuffer(target.files[0])
reader.onloadend = (e) => {
var data = new Uint8Array(e.target.result);
var workbook = XLSX.read(data, { type: 'array' });
workbook.SheetNames.forEach(function (sheetName) {
var XL_row_object = XLSX.utils.sheet_to_row_object_array(workbook.Sheets[sheetName]);
hojas.push({
data: XL_row_object,
sheetName
})
});
setExcel(hojas);
}
setLoading(false);
}
const sendDataToBackend = async (event) => {
event.preventDefault();
try {
if (emailNotification === undefined || emailNotification.trim() === '') {
swal("Ups!", `Email ${emailNotification} is not valid.`, "error");
return false;
}
if (emailNotification.includes(';')) {
const emails = emailNotification.split(';');
for (const email in emails) {
if (!EmailValidator.validate(emails[email])) {
swal("Ups!", `Email ${emails[email]} is not valid.`, "error");
return false;
}
}
}
if (excel === undefined || excel === null) {
swal("Ups!", `There is not file to process.`, "error");
return false;
}
setLoading(true);
const result = await axios.post(`${props.baseUrl}/emission/la-polar/3/${emailNotification}`, excel);
if (result !== undefined)
swal("Bulk Emission", `File ${filename} is loading. You will be notified by email at ${emailNotification} .`, "success");
else
swal("Ups!", `There was an error. Try again.`, "error");
setExcel(undefined);
} catch (err) {
console.error(err);
swal("Ups!", `There was an error. Try again: ${err}`, "error");
return false;
} finally {
setLoading(false);
}
}
const handleEmailChange = (event) => {
event.preventDefault();
setEmailNotification(event.target.value);
}
return (
<div>
<LockedWindow show={loading} />
<div className="widget">
<div className="container-fluid">
<div className="title">Emission la Polar</div>
<div className="separator"></div>
<div className="separator_blank"></div>
<div className="row">
<div className="col-12">
<div className="form-group">
<label htmlFor="exampleInputEmail1">Notification Emails</label>
<input type="email" className="form-control" id="exampleInputEmail1"
onChange={handleEmailChange}
value={emailNotification}
placeholder="Your email here. You can put more than one separated by ; (semicolon)" />
</div>
</div>
</div>
<div className="row">
<div className="col-12">
<div className="custom-file">
<input
accept=".xlsx"
type="file"
className="custom-file-input"
id="customFileLang"
lang="es"
onChange={handleFileChange}
placeholder={filename} readOnly />
<label className="custom-file-label" htmlFor="customFileLang">{filename}</label>
</div>
</div>
</div>
<div className="row">
<div className="col-12">
<ExcelDataLayout excel={excel} />
</div>
</div>
<div className="row">
<div className="col-12 d-flex justify-content-end align-items-center">
<button type="button" className="btn btn-primary" onClick={sendDataToBackend}>Procesar</button>
</div>
</div>
</div>
</div>
</div>
);
}
export default EmissionLaPolar;
<file_sep>/src/components/Login/Login.js
import React from 'react';
import logo from '../../assets/img/logo.svg';
import './Login.css';
const Login = (props) => {
return (
<div className="container" >
<div className="row full-height d-flex justify-content-center align-items-center" >
<div className="col-10 col-md-4 d-flex justify-content-center align-items-center" >
<img src={logo} width="200px" alt="" />
</div>
<div className="col-10 col-md-4 login-card" >
<div className="form-group">
<label htmlFor="txt-username">User Name</label>
<input type="text" className="form-control" id="txt-username" name="username" onChange={props.onInputChange} />
</div>
<div className="form-group">
<label htmlFor="txt-password">Password</label>
<input type="<PASSWORD>" className="form-control" id="txt-password" name="password" onChange={props.onInputChange} />
</div>
<div className="btn-login">
<button type="button" className="btn btn-primary" onClick={props.onLoginClick}>Log In</button>
</div>
</div>
</div>
</div>
);
}
Login.propTypes = {};
Login.defaultProps = {};
export default Login;<file_sep>/src/App.js
import Login from './components/Login/Login';
import LockedWindow from './components/LockedWindow/LockedWindow';
import React, { useState } from 'react';
import axios from 'axios';
import Home from './components/Home/Home';
import swal from 'sweetalert';
axios.interceptors.request.use(
request => {
if (request.url.includes('login'))
return request;
request.headers['Authorization'] = `Bearer ${localStorage.getItem('jwt')}`;
return request;
},
error => {
swal("Ups!", `There was an error. Try again: ${error}`, "error");
return Promise.reject(error);
}
);
axios.interceptors.response.use(
response => {
return response;
},
error => {
swal("Ups!", `There was an error. Try again: ${error}`, "error");
return Promise.reject(error);
}
);
const App = () => {
const BASE_URL = 'http://localhost:8082';
const [login, setLogin] = useState({
username: '',
password: ''
});
const [loged, setLoged] = useState(false);
const [loading, setLoading] = useState(false);
const [menus, setMenus] = useState([]);
const [currentMenu, setCurrentMenu] = useState(undefined);
const handleLoginInputChange = (event) => {
setLogin({
...login,
[event.target.name]: event.target.value
})
}
const handleClickLogin = async (e) => {
e.preventDefault();
try {
if (login.username.trimEnd().trimStart() === '' || login.password.trimEnd().trimStart() === '')
return false;
setLoading(true);
const result = await axios.post(`${BASE_URL}/login`, login);
if (result === undefined) {
swal("Ups!", "Result of login is undefined", "error");
return false;
}
localStorage.setItem('jwt', result.data.data);
setMenus([
{
id: 1,
name: "Emission",
menus: [
{ id: 2, name: "Emission - La Polar" },
{ id: 3, name: "<NAME>" }
]
}
]);
setLoged(true);
} catch (err) {
console.error(err);
swal("Ups!", `There was an error. Try again: ${err}`, "error");
setLogin({
username: '',
password: ''
});
} finally {
setLoading(false);
}
}
const handleClickLogout = (event) => {
event.preventDefault();
setLoading(true);
setLoged(false);
setLoading(false);
}
const handleClickMenuOption = (menu) => (event) => {
event.preventDefault();
setCurrentMenu(menu);
}
if (loading)
return (<LockedWindow show={loading} />);
if (!loged)
return (
<div>
<Login onLoginClick={handleClickLogin} login={login} onInputChange={handleLoginInputChange} />
</div>
);
return (
<div>
<Home
baseUrl={BASE_URL}
loading={loading}
onClickLogout={handleClickLogout}
username={login.username}
menus={menus}
onclickMenuOption={handleClickMenuOption}
currentMenu={currentMenu} />
</div>);
}
export default App; | 49eb7fde467b02a902a7ae8dc206e0a20d8205ce | [
"JavaScript"
] | 5 | JavaScript | yeti-iasof/bx-integration | f58377b171e9975d6d9adde09ba8d6b4a445c8fd | ce8a1d82b9004363e34a2029ae7bbc2ac3f505a9 | |
refs/heads/master | <file_sep>package org.opensource.analysis.byclass;
import org.objectweb.asm.ClassReader;
import org.objectweb.asm.ClassVisitor;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.net.URLClassLoader;
import java.nio.file.Paths;
import java.util.List;
public class ClassCallGraph {
public static void main(String[] args) throws IOException {
URL url = Paths.get("/Users/lvtu/workspace/java-callgraph/target/classes").toUri().toURL();
ClassLoader cl = new URLClassLoader(new URL[]{url});
InputStream ins = cl.getResourceAsStream("org/opensource/analysis/bysource/SourceCallGraph.class");
ClassReader cr = new ClassReader(ins);
ClassVisitor cw = new ClassCallVisitor();
cr.accept(cw, ClassReader.EXPAND_FRAMES);
}
}
<file_sep>package org.opensource.analysis.parse;
public interface ICallGraph {
void callGraph(String className, String method);
void search(String className, String method);
}
<file_sep>package org.opensource.analysis.parse.graph;
public interface MethodEdge {
}
<file_sep>package org.opensource.analysis.byclass;
import org.objectweb.asm.MethodVisitor;
import org.objectweb.asm.Opcodes;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MethodCallVisitor extends MethodVisitor {
private final static Logger logger = LoggerFactory.getLogger(MethodCallVisitor.class);
public MethodCallVisitor() {
super(Opcodes.ASM7);
}
@Override
public void visitMethodInsn(int opcode, String owner, String name, String descriptor, boolean isInterface) {
if (name.equals("<init>") || owner.startsWith("java")) {
super.visitMethodInsn(opcode, owner, name, descriptor, isInterface);
return;
}
logger.info("-> {}.{}", owner, name);
}
@Override
public void visitCode() {
super.visitCode();
}
@Override
public void visitMaxs(int maxStack, int maxLocals) {
super.visitMaxs(maxStack, maxLocals);
}
}
<file_sep>package org.opensource.analysis.parse.structure;
import org.objectweb.asm.Opcodes;
public class MethodrefInfo {
private int opcode;
private String owner;
private String name;
private String descriptor;
private boolean isInterface;
private boolean resolvedResult;
public MethodrefInfo(int opcode, String owner, String name, String descriptor, boolean isInterface) {
this.opcode = opcode;
this.owner = owner;
this.name = name;
this.descriptor = descriptor;
this.isInterface = isInterface;
}
public boolean isInvokeVirtual() {
return opcode == Opcodes.INVOKEVIRTUAL;
}
public boolean isInvokeInterface() {
return opcode == Opcodes.INVOKEINTERFACE;
}
public boolean isInvokeSpecial() {
return opcode == Opcodes.INVOKESPECIAL;
}
public boolean isInvokeStatic() {
return opcode == Opcodes.INVOKESTATIC;
}
public boolean isInvokeDynamic() {
return opcode == Opcodes.INVOKEDYNAMIC;
}
public int getOpcode() {
return opcode;
}
public String getOwner() {
return owner;
}
public String getName() {
return name;
}
public String getDescriptor() {
return descriptor;
}
public boolean isResolvedResult() {
return resolvedResult;
}
public void setResolvedResult(boolean resolvedResult) {
this.resolvedResult = resolvedResult;
}
}
<file_sep>package org.opensource.analysis.parse;
import org.apache.commons.lang3.StringUtils;
import org.opensource.analysis.parse.structure.ClassInfo;
import org.opensource.analysis.parse.structure.MethodInfo;
import org.opensource.analysis.parse.structure.MethodrefInfo;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.*;
public class ClassMethodGraph implements ICallGraph {
private final static Logger logger = LoggerFactory.getLogger(ClassMethodGraph.class);
private GraphClassResolver graphClassResolver;
public ClassMethodGraph(GraphClassResolver graphClassResolver) {
this.graphClassResolver = graphClassResolver;
}
public static final ThreadLocal<List> stack = ThreadLocal.withInitial(ArrayList::new);
@Override
public void callGraph(String className, String method) {
Map<String, ClassInfo> resolvedClassesMap = graphClassResolver.getResolvedTable().getResolvedClassesMap();
ClassInfo classInfo = resolvedClassesMap.get(StringUtils.replace(className, ".", "/"));
if (Objects.isNull(classInfo)) {
//可能没有解析成功,再解析一遍
if (!graphClassResolver.resolveClass(className)) {
//仍然没有解析成功
return;
}
}
classInfo = resolvedClassesMap.get(StringUtils.replace(className, ".", "/"));
//调用栈并且递归解析
Optional<MethodInfo> methodInfoOptional = classInfo.getMethods()
.stream()
.filter(methodInfo -> methodInfo.getName().equals(method))
.findFirst();
List<MethodrefInfo> methodrefInfos = methodInfoOptional.get().getMethodrefInfos();
for (int i = 0; i < methodrefInfos.size(); i++) {
MethodrefInfo methodrefInfo = methodrefInfos.get(i);
logger.info("called class:{},method:{}", methodrefInfo.getOwner(), methodrefInfo.getName());
callGraph(methodrefInfo.getOwner(), methodrefInfo.getName());
}
}
@Override
public void search(String className, String method) {
}
}
<file_sep>package org.opensource.analysis.algorithms;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.concurrent.atomic.AtomicLong;
import java.util.concurrent.atomic.LongAdder;
/**
* 原子命令性能评估
* 1亿累加:
* atom:620ms
* 普通(带寄存器优化):7ms,差距88倍
*/
public class CasPerformance {
public static void main(String[] args) throws InterruptedException {
// test();
// long start = System.currentTimeMillis();
// AtomicInteger atom = new AtomicInteger();
// for (int i = 0; i < 10000 * 10000; i++) {
// atom.incrementAndGet();
// }
// long end = System.currentTimeMillis();
// System.out.println(end - start + "ms");
// test();
test2();
// test3();
test4();
}
public static void test() {
long start = System.currentTimeMillis();
long local = 0;
for (int i = 0; i < 10000 * 10000; i++) {
++local;
}
long end = System.currentTimeMillis();
System.out.println(local);
System.out.println(end - start + "ms");
}
/**
*
*/
public static void test2() throws InterruptedException {
ExecutorService executorService = Executors.newFixedThreadPool(64);
LongAdder base = new LongAdder();
long start = System.currentTimeMillis();
for (int i = 0; i < 2000 * 10000; i++) {
executorService.submit(base::increment);
}
executorService.shutdown();
executorService.awaitTermination(1, TimeUnit.HOURS);
long end = System.currentTimeMillis();
System.out.println("LongAdder :" + (end - start));
}
public static void test3() throws InterruptedException {
ExecutorService executorService = Executors.newFixedThreadPool(500);
AtomicInteger base = new AtomicInteger();
long start = System.currentTimeMillis();
for (int i = 0; i < 2000 * 10000; i++) {
executorService.submit(base::incrementAndGet);
}
executorService.shutdown();
executorService.awaitTermination(1, TimeUnit.HOURS);
long end = System.currentTimeMillis();
System.out.println("AtomicInteger :" + (end - start));
}
public static void test4() throws InterruptedException {
ExecutorService executorService = Executors.newFixedThreadPool(64);
AtomicLong base = new AtomicLong();
long start = System.currentTimeMillis();
for (int i = 0; i < 2000 * 10000; i++) {
executorService.submit(base::incrementAndGet);
}
executorService.shutdown();
executorService.awaitTermination(1, TimeUnit.HOURS);
long end = System.currentTimeMillis();
System.out.println("AtomicLong :" + (end - start));
}
}
<file_sep>```text
1.sp callgraph -cp [classpath] package.class.method
package.class.method
->package.class.method
->package.class.method
->package.class.method
->package.class.method
->package.class.method
package.class.method
->package.class.method
->package.class.method
2.sp search -cp [classpath] package.class.method
package.class.method
<-package.class.method
<-package.class.method
<-package.class.method
<-package.class.method
<-package.class.method
<-package.class.method
<-package.class.method
<-package.class.method
callgraph实现方法:
例如package.classA.methodB
1.find class:package.classA
2.find All methods of A
2-1.save all call instructions
3.find specified methodB from step2
4.for each insn in methodB
repeat step-1
5.end until some call terminal methods
analysis:
ClassResolver.resolveClass(class)
ClassVisitor
MethodResolver.resolveMethod(method) => ClassResolver.resolveClass(class) => MethodResolver.resolveMethod(method)
MethodVisitor
ClassLoader
ResolvedClassTable
ResolvedMethodTable
CallGraph:method --ref--> method
怎样表达两个方法的引用关系,或者说用怎样的数据结构描绘这个graph?
methodA
ref call-ref
ref methodB
public <T,K> T void(K parameter);
```
```text
analyzer
1.class-resolver:读取class文件,解析类和方法----创建MethodNode
2.method-resolver:读取method code,解析被调用方法的类和方法----先解析类和方法,再根据语言特性继续解析可能的父类和接口类
step-1和step-2把文件读取到内存中,把文件中的结构在内存中再组织一遍:
文件中的结构:调用关系隐式存在;
内存中的结构:调用关系,类和方法的组织关系,类的属性,方法的属性等等,在内存中显式存储,并可以对外暴露接口使用。
3.graph:利用前面步骤的接口,建立graph接口,可供搜索遍历
实际的顺序可能会被调整,即可能graph3 -> class1 -> method2 -> class1 ->method2 这个互相插入的的顺序
说明:
这几个步骤的目的都是为了使结构structure使用起来更加方便,因为需要不同,组织结构的方式也不同,接口提供的功能也不相同。
基本元素是相同,客观存在的关系是相同的,但是对于不同的要求层次,组织和使用的方式却是不同的,为了让使用起来更加方便,抽象了以上概念。
即使元素只有0和1,但是也能组合成不同的形式,也就需要层次抽象来帮助管理。
```
```text
resolve ref伪代码:
function callgraph(ref)
resolveMethod(ref)=>class+method+ref_list
for each ref in ref_list
callgraph(ref)
为了简单化,处理如下:
1.如果遇到接口interface,不再继续resolve,后续支持;
2.接口调用可能会形成死循环,这时要break死循环;
难点:
1.接口没有body,需要找到实现impl; (findDirectImplClasses,findAllImplClasses)
2.类有子类,使用哪一个实现; (findDirectSubClasses,findAllSubClasses)
3.怎样避免cycle调用;
4.怎样清楚优雅地显示;
5.多态节点的展示方法和数据结构
interface:
findDirectSubInterfaces(parameter:interface)
findSubInterfaces(parameter:interface)
findDirectImplClasses(parameter:interface)
findAllImplClasses(parameter:interface)
impl class:
findDirectSubClasses(parameter:impl class)
findAllSubClasses(parameter:impl class)
invokeinterface:findDirectImplClasses,findAllImplClasses
invokevirtual:findDirectSubClasses,findAllSubClasses
invokestatic:
invokespecial:
invokedynamic:略
类的关系
resolve和classloader是一对一的关系
不同的classloader会单独加载class,但是resolve的逻辑是一样的
需要补充的算法知识:
1.环路检测:利用stack追踪调用链,检测环路
2.图的表达:数据结构
3.图的展示
4.图的遍历
```<file_sep>package org.opensource.analysis.parse.graph;
import java.util.List;
public interface MethodNode {
/**
* 根据method ref发现真实的方法节点,如果不能发现,那么就创造一个虚拟节点
*
* @return
*/
List<MethodNode> findRefNodes();
List<MethodNode> possibleMethodNodes();
boolean existsMultiImpl();
}
<file_sep>package org.opensource.analysis.parse.structure;
import java.util.ArrayList;
import java.util.List;
public class MethodInfo {
private ClassInfo ownerClassInfo;
private int access;
private String name;
private String descriptor;
private String signature;
private String[] exceptions;
public MethodInfo(int access, String name, String descriptor, String signature, String[] exceptions) {
this.access = access;
this.name = name;
this.descriptor = descriptor;
this.signature = signature;
this.exceptions = exceptions;
}
private List<MethodrefInfo> methodrefInfos = new ArrayList<>();
public void addMethodRef(MethodrefInfo methodref) {
methodrefInfos.add(methodref);
}
public ClassInfo getOwnerClassInfo() {
return ownerClassInfo;
}
public void setOwnerClassInfo(ClassInfo ownerClassInfo) {
this.ownerClassInfo = ownerClassInfo;
}
public int getAccess() {
return access;
}
public String getName() {
return name;
}
public String getDescriptor() {
return descriptor;
}
public String getSignature() {
return signature;
}
public String[] getExceptions() {
return exceptions;
}
public List<MethodrefInfo> getMethodrefInfos() {
return methodrefInfos;
}
}
<file_sep>package org.opensource.analysis.bysource;
import org.antlr4.java.JavaBaseVisitor;
import org.antlr4.java.JavaParser;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MethodVisitor extends JavaBaseVisitor {
private final static Logger logger = LoggerFactory.getLogger(MethodVisitor.class);
@Override
public Object visitMethodDeclaration(JavaParser.MethodDeclarationContext ctx) {
logger.info("visitMethodDeclaration:{}", ctx.getText());
return super.visitMethodDeclaration(ctx);
}
@Override
public Object visitMethodModifier(JavaParser.MethodModifierContext ctx) {
return super.visitMethodModifier(ctx);
}
@Override
public Object visitMethodHeader(JavaParser.MethodHeaderContext ctx) {
logger.info("visitMethodHeader:{}", ctx.getText());
return super.visitMethodHeader(ctx);
}
@Override
public Object visitMethodName(JavaParser.MethodNameContext ctx) {
return super.visitMethodName(ctx);
}
@Override
public Object visitMethodDeclarator(JavaParser.MethodDeclaratorContext ctx) {
return super.visitMethodDeclarator(ctx);
}
@Override
public Object visitMethodBody(JavaParser.MethodBodyContext ctx) {
logger.info("visitMethodBody:{}", ctx.getText());
return super.visitMethodBody(ctx);
}
@Override
public Object visitMethodInvocation(JavaParser.MethodInvocationContext ctx) {
logger.info("visitMethodInvocation:{}", ctx.getText());
return super.visitMethodInvocation(ctx);
}
@Override
public Object visitInterfaceMethodDeclaration(JavaParser.InterfaceMethodDeclarationContext ctx) {
return super.visitInterfaceMethodDeclaration(ctx);
}
@Override
public Object visitInterfaceMethodModifier(JavaParser.InterfaceMethodModifierContext ctx) {
return super.visitInterfaceMethodModifier(ctx);
}
@Override
public Object visitMethodReference(JavaParser.MethodReferenceContext ctx) {
logger.info("visitMethodReference:{}", ctx.getText());
return super.visitMethodReference(ctx);
}
@Override
public Object visitMethodReference_lf_primary(JavaParser.MethodReference_lf_primaryContext ctx) {
return super.visitMethodReference_lf_primary(ctx);
}
@Override
public Object visitMethodReference_lfno_primary(JavaParser.MethodReference_lfno_primaryContext ctx) {
return super.visitMethodReference_lfno_primary(ctx);
}
@Override
public Object visitMethodInvocation_lf_primary(JavaParser.MethodInvocation_lf_primaryContext ctx) {
return super.visitMethodInvocation_lf_primary(ctx);
}
@Override
public Object visitMethodInvocation_lfno_primary(JavaParser.MethodInvocation_lfno_primaryContext ctx) {
return super.visitMethodInvocation_lfno_primary(ctx);
}
}
<file_sep>package org.opensource.analysis.parse;
import org.objectweb.asm.MethodVisitor;
import org.objectweb.asm.Opcodes;
import org.opensource.analysis.parse.structure.ClassInfo;
import org.opensource.analysis.parse.structure.MethodInfo;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class GraphClassVisitor extends org.objectweb.asm.ClassVisitor {
private final static Logger logger = LoggerFactory.getLogger(GraphClassVisitor.class);
private ClassInfo classInfo;
private GraphClassResolver classResolver;
public GraphClassVisitor(GraphClassResolver classResolver) {
super(Opcodes.ASM7);
this.classResolver = classResolver;
}
@Override
public void visit(int version, int access, String name, String signature, String superName, String[] interfaces) {
classInfo = new ClassInfo(access, name, signature, superName, interfaces);
super.visit(version, access, name, signature, superName, interfaces);
}
@Override
public MethodVisitor visitMethod(int access, String name, String descriptor, String signature, String[] exceptions) {
MethodInfo methodInfo = new MethodInfo(access, name, descriptor, signature, exceptions);
classInfo.addMethod(methodInfo);
return new GraphMethodVisitor(methodInfo);
}
@Override
public void visitEnd() {
super.visitEnd();
classResolver.getResolvedTable().addClass(classInfo);
}
}
<file_sep>package org.opensource.analysis.parse.graph;
import java.util.List;
public abstract class AbstractMethodNode implements MethodNode{
@Override
public List<MethodNode> findRefNodes() {
return null;
}
@Override
public List<MethodNode> possibleMethodNodes() {
return null;
}
@Override
public boolean existsMultiImpl() {
return false;
}
}
<file_sep>package org.opensource.analysis.parse;
import org.opensource.analysis.parse.structure.MethodrefInfo;
public class GraphMethodResolver {
private GraphClassResolver classResolver;
public GraphMethodResolver(GraphClassResolver classResolver) {
this.classResolver = classResolver;
}
public void resolveMethodref(MethodrefInfo methodrefInfo) {
boolean resolvedResult = classResolver.resolveClass(methodrefInfo.getOwner());
methodrefInfo.setResolvedResult(resolvedResult);
if (methodrefInfo.isInvokeInterface()) {
//寻找实现
} else if (methodrefInfo.isInvokeSpecial()) {
//寻找所有实现类及子类
} else if (methodrefInfo.isInvokeVirtual()) {
//寻找所有实现类及子类
} else if (methodrefInfo.isInvokeStatic()) {
//寻找所有实现类及子类
} else if (methodrefInfo.isInvokeDynamic()) {
}
}
}
<file_sep>package org.opensource.analysis.parse.graph;
import java.util.List;
/**
* 多态节点,因为面向对象:封装,继承,多态的特性,可以理解为所有的节点都是多态节点
*/
public class PolymorphismNode extends AbstractMethodNode {
private List<MethodNode> possibleNodes;
@Override
public List<MethodNode> findRefNodes() {
return super.findRefNodes();
}
@Override
public List<MethodNode> possibleMethodNodes() {
return possibleNodes;
}
@Override
public boolean existsMultiImpl() {
return true;
}
}
<file_sep>package org.opensource.analysis.bysource;
import org.antlr.v4.runtime.CharStream;
import org.antlr.v4.runtime.CharStreams;
import org.antlr.v4.runtime.CommonTokenStream;
import org.antlr4.java.JavaLexer;
import org.antlr4.java.JavaParser;
import java.io.IOException;
import java.nio.charset.Charset;
public class SourceCallGraph {
public static void main(String[] args) throws IOException {
String sFile = "/Users/lvtu/workspace/java-callgraph/src/main/java/org/opensource/analysis/CallGraph.java";
CharStream cs = CharStreams.fromFileName(sFile, Charset.forName("utf-8"));
JavaLexer lexer = new JavaLexer(cs);
CommonTokenStream tokens = new CommonTokenStream(lexer);
JavaParser parser = new JavaParser(tokens);
JavaParser.CompilationUnitContext tree = parser.compilationUnit(); // parse a compilationUnit
int hashCode = cs.hashCode();
System.out.println(hashCode);
MethodVisitor methodVisitor = new MethodVisitor();
tree.accept(methodVisitor);
selfCall();
}
private static void selfCall() {
}
}
| d5e87effe68527e8d8284dee0948082ef9168f24 | [
"Markdown",
"Java"
] | 16 | Java | buzhidaolvtu/java-callgraph | 221c997128a320d1c19a932589468f90ec1cbbb5 | defdd712f09b7c1936f1ff4bb525e38902119670 | |
refs/heads/master | <file_sep><?php
/* 功能不完善,后续根据开发需要,慢慢完善,替换以前系统底层 */
/* 一步一步替换掉 */
/**
* 定义根目录
**/
define('TS_ROOT', dirname(__FILE__) . DIRECTORY_SEPARATOR . '..' . DIRECTORY_SEPARATOR);
include dirname(__FILE__) . DIRECTORY_SEPARATOR . 'Ts.php';
Ts::run(); | bae01158a82ac03fe7212319585b56dc839f5487 | [
"PHP"
] | 1 | PHP | pf5512/ThinkSNS-4 | 6d22efb2b8aee0ea200431168af29320ac0d461d | 774123dd0688fed397b1c385fbbbdbf9ef11a9c2 | |
refs/heads/master | <file_sep># Another google apps engine go logger this one is for the new go111+ world..
Usage is simple:
```go
import (
"github.com/arran4/gaelogger"
"net/http"
)
func init() {
http.HandleFunc("/", handle)
}
func handle(writer http.ResponseWriter, request *http.Request) {
logger := gaelogger.NewLogger(request)
defer logger.Close()
logger.Infof("%s request: %v %v", request.Method, request.URL.RawPath, request.URL.RawQuery)
}
```
<file_sep>module github.com/arran4/gaelogger
go 1.20
require (
cloud.google.com/go/logging v1.7.0
github.com/golang/groupcache v0.0.0-20210331224755-41bb18bfe9da // indirect
golang.org/x/oauth2 v0.6.0 // indirect
google.golang.org/api v0.111.0 // indirect
google.golang.org/genproto v0.0.0-20230306155012-7f2fa6fef1f4 // indirect
)
<file_sep>package gaelogger
import (
"cloud.google.com/go/logging"
"context"
"fmt"
"io"
"log"
"net/http"
"os"
"runtime"
)
type backLogger interface {
io.Closer
logf(severity fmt.Stringer, format string, args ...interface{})
}
type Logger interface {
io.Closer
Defaultf(format string, args ...interface{})
Debugf(format string, args ...interface{})
Infof(format string, args ...interface{})
Noticef(format string, args ...interface{})
Warningf(format string, args ...interface{})
Errorf(format string, args ...interface{})
Criticalf(format string, args ...interface{})
Alertf(format string, args ...interface{})
Emergencyf(format string, args ...interface{})
}
func NewLogger(r *http.Request) Logger {
if len(os.Getenv("GOOGLE_CLOUD_PROJECT")) > 0 {
var ctx context.Context
if r != nil {
ctx = r.Context()
} else {
ctx = context.Background()
}
loggingClient, err := logging.NewClient(ctx, os.Getenv("GOOGLE_CLOUD_PROJECT"))
if err != nil {
log.Fatalf("Failed to create client: %v", err)
}
logger := loggingClient.Logger("request")
return &WrapLogger{
backLogger: &GaeLogger{
loggingClient: loggingClient,
logger: logger,
r: r,
},
}
} else {
return &WrapLogger{
backLogger: &StdLogger{
logger: log.New(os.Stderr, "", log.Lshortfile|log.LstdFlags),
},
}
}
}
type StdLogger struct {
logger *log.Logger
}
func (st *StdLogger) Close() error {
return nil
}
func (st *StdLogger) logf(severity fmt.Stringer, format string, args ...interface{}) {
st.logger.SetPrefix(severity.String()+" ")
st.logger.Output(3, fmt.Sprintf(format, args...))
st.logger.SetPrefix("")
}
type GaeLogger struct {
loggingClient *logging.Client
logger *logging.Logger
r *http.Request
}
func (gl *GaeLogger) Close() error {
if gl.loggingClient != nil {
return gl.loggingClient.Close()
}
return nil
}
func (gl *GaeLogger) logf(severity fmt.Stringer, format string, args ...interface{}) {
_, file, line, ok := runtime.Caller(2)
if !ok {
file = "???"
line = 0
}
gl.logger.Log(logging.Entry{
Severity: severity.(logging.Severity),
Payload: fmt.Sprintf("%s:%d "+format, append([]interface{}{file, line}, args...)...),
HTTPRequest: &logging.HTTPRequest{
Request: gl.r,
},
})
}
type WrapLogger struct {
backLogger
}
func (gl WrapLogger) Defaultf(format string, args ...interface{}) {
gl.logf(logging.Default, format, args...)
}
func (gl *WrapLogger) Debugf(format string, args ...interface{}) {
gl.logf(logging.Debug, format, args...)
}
func (gl *WrapLogger) Infof(format string, args ...interface{}) {
gl.logf(logging.Info, format, args...)
}
func (gl *WrapLogger) Noticef(format string, args ...interface{}) {
gl.logf(logging.Notice, format, args...)
}
func (gl *WrapLogger) Warningf(format string, args ...interface{}) {
gl.logf(logging.Warning, format, args...)
}
func (gl *WrapLogger) Errorf(format string, args ...interface{}) {
gl.logf(logging.Error, format, args...)
}
func (gl *WrapLogger) Criticalf(format string, args ...interface{}) {
gl.logf(logging.Critical, format, args...)
}
func (gl *WrapLogger) Alertf(format string, args ...interface{}) {
gl.logf(logging.Alert, format, args...)
}
func (gl *WrapLogger) Emergencyf(format string, args ...interface{}) {
gl.logf(logging.Emergency, format, args...)
}
| c84c87960f2156e7eb4a06a8a606549eabadbe8f | [
"Markdown",
"Go Module",
"Go"
] | 3 | Markdown | arran4/gaelogger | 0a6f9ba7c16a057d0680f07691b67f584835bf89 | 524c4d7025410d0caf18b419470426e08ad7b630 | |
refs/heads/master | <repo_name>gizeminthecity/lab-react-training<file_sep>/src/components/ClickablePicture.jsx
import React from 'react';
function ClickablePicture(props) {
const [picture, setPicture] = React.useState('false');
const handleClick = () => setPicture(!picture);
return (
<div>
<img
onClick={handleClick}
src={!picture ? props.imgClicked : props.img}
alt="max"
/>
</div>
);
}
export default ClickablePicture;
<file_sep>/src/components/Dice.jsx
import React from 'react';
function Dice() {
const [dice, setDice] = React.useState('3');
const handleClick = () => {
const randomDice = Math.floor(Math.random() * 6) + 1;
setDice('-empty');
setTimeout(() => {
setDice(randomDice);
}, 1000);
return dice;
};
return (
<div>
<img
style={{ width: '200px' }}
onClick={() => handleClick()}
src={`/img/dice${dice}.png`}
/>
</div>
);
}
export default Dice;
<file_sep>/src/components/Facebook.jsx
import React from 'react';
import profiles from '../data/berlin.json';
function Facebook() {
return (
<div>
{profiles.map((el) => {
return (
<div
style={{
border: '1px solid black',
display: 'flex',
margin: '2rem',
}}
>
<div>
<img style={{ width: '150px' }} src={el.img} />
</div>
<div>
<p>First name: {el.firstName}</p>
<p>Last name: {el.lastName}</p>
<p>County: {el.country}</p>
<p>Type: {el.isStudent ? 'Student' : 'Teacher'}</p>
</div>
</div>
);
})}
</div>
);
}
export default Facebook;
<file_sep>/src/components/CreditCard.jsx
import React from 'react';
function CreditCard(props) {
const {
type,
number,
expirationMonth,
expirationYear,
bank,
owner,
bgColor,
color,
} = props;
const digits = number.slice(-4);
const cardType = {
Visa: '/img/visa.png',
'Master Card': '/img/master-card.svg',
};
const cardColor = {
backgroundColor: bgColor,
color,
};
return (
<div style={cardColor}>
<div>
<img src={cardType[type]} />
</div>
<div>
<p>
{'*'.repeat(12)} {digits}
</p>
</div>
<div>
Expires: {expirationMonth > 9 ? expirationMonth : `0${expirationMonth}`}{' '}
/ {expirationYear - 2000} - {bank}
</div>
<div>{owner}</div>
</div>
);
}
export default CreditCard;
<file_sep>/src/components/LikeButton.jsx
import React from 'react';
function LikeButton(props) {
const colors = ['purple', 'blue', 'green', 'yellow', 'orange', 'red'];
const [counter, setCounter] = React.useState(0);
const handleLike = () => {
return setCounter(counter + 1);
};
return (
<div>
<button
onClick={handleLike}
style={{
background: `${colors[counter]}`,
}}
>
{counter} Likes
</button>
</div>
);
}
export default LikeButton;
<file_sep>/src/components/Carousel.jsx
import React from 'react';
function Carousel(props) {
const [counter, setCounter] = React.useState(0);
const handleRight = () => (counter < 3 ? setCounter(counter + 1) : counter);
const handleLeft = () => (counter > 0 ? setCounter(counter - 1) : counter);
return (
<div>
<button onClick={handleLeft}>Left</button>
<img src={props.imgs[counter]} />
<button onClick={handleRight}>Right</button>
</div>
);
}
export default Carousel;
<file_sep>/src/components/NumbersTable.jsx
import React from 'react';
function NumbersTable(props) {
const numbers = [];
for (let i = 1; i <= props.limit; i++) numbers.push(i);
return (
<div style={{ display: 'flex', margin: '5rem' }}>
{numbers.map((el) => {
return el % 2 === 0 ? (
<div
style={{
width: '150px',
height: '50px',
border: '1px solid black',
backgroundColor: 'red',
}}
>
<p>{el}</p>
</div>
) : (
<div
style={{
width: '150px',
height: '50px',
border: '1px solid black',
}}
>
<p>{el}</p>
</div>
);
})}
</div>
);
}
export default NumbersTable;
| 5fe6c54ec0504cbed44104d6dd9516e6328804e4 | [
"JavaScript"
] | 7 | JavaScript | gizeminthecity/lab-react-training | 117297eed2dee050b9edbddda104c27b578850eb | 48f4132b7bd5740a09570629e098974be9b7f9b3 | |
refs/heads/master | <repo_name>wtrb/go<file_sep>/codility/10-prime-composite-numbers/min-perimeter-rectangle/min_perimeter_rectangle.go
package main
import (
"fmt"
)
// https://app.codility.com/demo/results/trainingZHTSZ9-M3D/
// Detected time complexity: O(sqrt(N))
func min(N int) int {
a := 1
b := N / a
minPeri := 2 * (a + b)
for a*a <= N {
if N%a == 0 {
b = N / a
cur := 2 * (a + b)
if cur < minPeri {
minPeri = cur
}
}
a++
}
return minPeri
}
func main() {
fmt.Println(min(30)) // 22
}
<file_sep>/tour/others/ellipsis.go
package main
import (
"fmt"
)
func main() {
// 1. variadic parameter
fmt.Println(Sum(2, 3, 6))
// 2.
primes := []int{2, 3, 5, 7}
fmt.Println(Sum(primes...)) // unpacking
// 3. Array literals
// the ... notation specifies a length equal to the number of elements in the literal
stooges := [...]string{"Moe", "Larry", "Curly"}
fmt.Println(len(stooges))
}
// Variadic function parameters
func Sum(nums ...int) int {
res := 0
for _, n := range nums {
res += n
}
return res
}
// Three dots<file_sep>/codility/06-sorting/disc-intersections/disc_test.go
package disc
import (
"testing"
)
func TestSolution(t *testing.T) {
for _, c := range testCases {
output := Solution(c.input)
if output != c.expected {
t.Fatalf("\nFAIL: %v\nInput: %v\nexpected: %v\ngot: %v", c.description, c.input, c.expected, output)
}
t.Logf("PASS: %v", c.description)
}
}
func BenchmarkSolution(b *testing.B) {
for i := 0; i < b.N; i++ {
for _, c := range testCases {
Solution(c.input)
}
}
}
var testCases = []struct {
description string
input []int
expected int
}{
{
description: "No.1",
input: []int{1, 5, 2, 1, 4, 0},
expected: 11,
},
}
<file_sep>/exercism/two-fer/two_fer.go
// Package twofer implements "One for you and one for me." logic.
package twofer
import "fmt"
// ShareWith returns the string "One for {name}, one for me.". If name is empty, "you" is used.
func ShareWith(name string) string {
if name == "" {
name = "you"
}
return fmt.Sprintf("One for %s, one for me.", name)
}
<file_sep>/tour/basic/08-pointer.go
package main
import (
"fmt"
)
func main() {
i := 7
fmt.Println("Memory address: ", &i)
inc1(i)
fmt.Println("i not modified: ", i)
inc2(&i)
fmt.Println("i incremented successfully: ", i)
fmt.Println("i memory address after incremented: ", &i, " , still the same")
}
func inc1(x int) {
x++
}
func inc2(x *int) {
*x++
}<file_sep>/tour/methods-interfaces/12-readers.go
package main
import (
"fmt"
"io"
"strings"
)
func main() {
r := strings.NewReader("Hello, Reader!")
b := make([]byte, 8)
for {
n, err := r.Read(b)
fmt.Printf("n = %v || err = %v || b = %v\n", n, err, b)
fmt.Printf("b[:n] = %q\n", b[:n])
fmt.Printf("---\n")
if err == io.EOF {
break
}
}
}
/*
n = 8 || err = <nil> || b = [72 101 108 108 111 44 32 82]
b[:n] = "Hello, R"
---
n = 6 || err = <nil> || b = [101 97 100 101 114 33 32 82]
b[:n] = "eader!"
---
n = 0 || err = EOF || b = [101 97 100 101 114 33 32 82]
b[:n] = ""
---
*/
/*
The io package specifies the io.Reader interface, which represents the read end of a stream of data.
The Go standard library contains many implementations of these interfaces, including files, network connections, compressors, ciphers, and others.
The io.Reader interface has a Read method:
func (T) Read(b []byte) (n int, err error)
*/<file_sep>/codility/09-max-slice-problem/max_slice_problem.go
package main
import (
"fmt"
)
// prefix sums
// O(n**2)
func max1(A []int) int {
prefixSums := make([]int, len(A)+1)
for i := 1; i < len(A)+1; i++ {
prefixSums[i] = prefixSums[i-1] + A[i-1]
}
maxSum := 0
for p := 0; p < len(A); p++ {
for q := p; q < len(A); q++ {
sum := prefixSums[q+1] - prefixSums[p]
if sum > maxSum {
maxSum = sum
}
}
}
return maxSum
}
// without prefix sums
// O(n**2)
func max2(A []int) int {
maxSum := 0
for p := 0; p < len(A); p++ {
sum := 0
for q := p; q < len(A); q++ {
sum += A[q]
if sum > maxSum {
maxSum = sum
}
}
}
return maxSum
}
// O(n)
func max(A []int) int {
maxSum := 0
sum := 0
for _, a := range A {
if sum+a > 0 {
sum += a
} else {
sum = 0
}
if sum > maxSum {
maxSum = sum
}
}
return maxSum
}
func main() {
fmt.Println(max([]int{1, 2, 3, 4, 5})) // 15
fmt.Println(max([]int{5, -7, 3, 5, -2, 4, -1})) // 10
fmt.Println(max([]int{5, -1})) // 5
}
<file_sep>/codility/others/prefix-multiply/prefix_multiply.go
package main
import (
"fmt"
)
func mul(A []int) []int {
result := make([]int, len(A))
for i := 1; i < len(A); i++ {
result[i-1] =
}
return result
}
func main() {
fmt.Println(mul([]int {1, 2, 3, 4}))
// [24, 12, 8, 6]
}<file_sep>/codility/05-prefix-sums/count-div/count_div.go
package div
// 0 <= A ≤ B <= 2,000,000,000
// 1 <= K <= 2,000,000,000
func Solution(A int, B int, K int) int {
if A == 0 {
return B/K + 1 // +1 because 0 mod any number = 0
}
return B/K - (A-1)/K
}
// https://app.codility.com/demo/results/trainingAZHYCH-BKG/
// Detected time complexity:
// O(1)
<file_sep>/exercism/house/house.go
package house
import (
"bytes"
"strings"
)
var (
subjects = [...]string{"This", "that", "that", "that", "that", "that", "that", "that", "that", "that", "that", "that"}
verbs = [...]string{
"is",
"belonged to",
"kept",
"woke",
"married",
"kissed",
"milked",
"tossed",
"worried",
"killed",
"ate",
"lay in",
}
objects = [...]string{
"the house that Jack built",
"the malt",
"the rat",
"the cat",
"the dog",
"the cow with the crumpled horn",
"the maiden all forlorn",
"the man all tattered and torn",
"the priest all shaven and shorn",
"the rooster that crowed in the morn",
"the farmer sowing his corn",
"the horse and the hound and the horn",
}
)
// Verse returns a part of the song.
func Verse(depth int) string {
var buf bytes.Buffer
// First line
s := subjects[0]
v := verbs[0]
o := objects[depth-1]
line := strings.Join([]string{s, v, o}, " ")
buf.WriteString(line)
// Second line and more
for i := 1; i < depth; i++ {
buf.WriteString("\n")
s = subjects[i]
v = verbs[len(verbs)-depth+i]
o = objects[depth-1-i]
line = strings.Join([]string{s, v, o}, " ")
buf.WriteString(line)
}
buf.WriteString(".")
return buf.String()
}
// Song returns the entire song.
func Song() string {
var buf bytes.Buffer
for i := 1; i <= len(verbs); i++ {
buf.WriteString(Verse(i))
buf.WriteString("\n\n")
}
return strings.TrimSuffix(buf.String(), "\n\n")
}
<file_sep>/learn-go-with-tests/fundamentals/03-iteration/iteration.go
package iteration
// Repeat returns character repeated by input times.
func Repeat(c string, count int) string {
if count <= 0 {
return c
}
var repeated string
for i := 0; i < count; i++ {
repeated += c
}
return repeated
}
<file_sep>/exercism/bank-account/bank_account.go
package account
import (
"sync"
)
// Account represents a bank account.
type Account struct {
sync.RWMutex
balance int64
closed bool
}
// Open opens an account with the initial deposit. A negative deposit returns nil.
func Open(initialDeposit int64) *Account {
if initialDeposit < 0 {
return nil
}
return &Account{balance: initialDeposit, closed: false}
}
// Close will close the account and return the current balance.
func (acc *Account) Close() (payout int64, ok bool) {
acc.Lock()
defer acc.Unlock()
if !acc.closed {
acc.closed = true
payout = acc.balance
ok = true
}
return
}
// Balance will return account's current balance, or (0, false) if the account is closed.
func (acc *Account) Balance() (balance int64, ok bool) {
acc.RLock()
defer acc.RUnlock()
if acc.closed {
return
}
return acc.balance, true
}
// Deposit will deposit or withdraw (if negative) the specified amount.
// Withdrawals will not succeed if they result in a negative balance.
func (acc *Account) Deposit(amount int64) (newBalance int64, ok bool) {
acc.Lock()
defer acc.Unlock()
if acc.closed {
return
}
newBalance = acc.balance + amount
if newBalance < 0 {
return
}
acc.balance = newBalance
ok = true
return
}
<file_sep>/tour/basic/07-function.go
package main
import (
"errors"
"fmt"
"math"
)
func main() {
// func
result := sum(233, 23)
fmt.Println("result = ", result)
//
result2, err := sqrt(-14)
if err != nil {
fmt.Println("err: ", err)
} else {
fmt.Println("square = ", result2)
}
}
// When two or more consecutive named function parameters share a type,
// you can omit the type from all but the last.
func sum(x, y int) int {
return x + y
}
// A function can return any number of results.
func sqrt(x float64) (float64, error) {
if x < 0 {
return 0, errors.New("Undefied for nagative numbers")
}
return math.Sqrt(x), nil
}
<file_sep>/exercism/robot-simulator/defs.go
package robot
// definitions used in step 1
// Step1Robot is robot.
var Step1Robot struct {
X, Y int
Dir
}
// Dir represents directions.
type Dir int
// Directions: North, East, South, West.
const (
N Dir = iota
E
S
W
)
// String returns formatted direction.
func (d Dir) String() (s string) {
switch d {
case N:
s = "North"
case E:
s = "East"
case S:
s = "South"
case W:
s = "West"
}
return
}
// Right turns right.
func Right() {
Step1Robot.Dir++
if Step1Robot.Dir > W {
Step1Robot.Dir = N
}
}
// Left turns left.
func Left() {
Step1Robot.Dir--
if Step1Robot.Dir < N {
Step1Robot.Dir = W
}
}
// Advance moves ahead.
func Advance() {
switch Step1Robot.Dir {
case N:
Step1Robot.Y++
case E:
Step1Robot.X++
case S:
Step1Robot.Y--
case W:
Step1Robot.X--
}
}
// additional definitions used in step 2
// Command commands the robot to move. Valid values are 'R', 'L', 'A'.
type Command byte
type RU int
type Pos struct{ Easting, Northing RU }
type Rect struct{ Min, Max Pos }
type Step2Robot struct {
Dir
Pos
}
// StartRobot starts robot.
func StartRobot(chan Command, chan Action) {
}
// Room prepares the room.
func Room(extent Rect, robot Step2Robot, act chan Action, rep chan Step2Robot) {
}
// additional definition used in step 3
// type Step3Robot struct {
// Name string
// Step2Robot
// }
<file_sep>/gophercises/README.md
# gophercises
gophercises.com
<file_sep>/exercism/bob/bob.go
// Package bob provides a function that simulates Bob's responses to people's questions.
package bob
import (
"strings"
)
// Remark is a convenience type for identifying what kind of remark it is.
type Remark string
func (r Remark) isQuestion() bool {
return strings.HasSuffix(string(r), "?")
}
func (r Remark) isYelling() bool {
allNonLetter := true
for _, c := range r {
if (c >= 'A' && c <= 'Z') || (c >= 'a' && c <= 'z') {
allNonLetter = false
}
if c >= 'a' && c <= 'z' {
return false
}
}
return !allNonLetter
}
func (r Remark) isExasperated() bool {
return r.isYelling() && r.isQuestion()
}
func (r Remark) isSilence() bool {
return r == ""
}
// Hey simulates Bob's reponse to inputted remark.
func Hey(remark string) string {
r := Remark(strings.TrimSpace(remark))
switch {
case r.isExasperated():
return "Calm down, I know what I'm doing!"
case r.isQuestion():
return "Sure."
case r.isYelling():
return "Whoa, chill out!"
case r.isSilence():
return "Fine. Be that way!"
default:
return "Whatever."
}
}
<file_sep>/codility/06-sorting/max-product/max_product.go
package product
import "sort"
// https://app.codility.com/demo/results/training3STRZ3-6SX/
// Detected time complexity:
// O(N * log(N))
func Solution(A []int) int {
sort.Ints(A)
i := len(A) - 1
rMax := A[i] * A[i-1] * A[i-2]
lMax := A[0] * A[1] * A[i]
if rMax > lMax {
return rMax
}
return lMax
}
// Time complexity: O(n)
// Space complexity: O(1)
func useSingleScan(nums []int) int {
min1, min2 := 1<<63-1, 1<<63-1
max1, max2, max3 := -1<<63, -1<<63, -1<<63
for _, n := range nums {
if n <= min1 {
min1, min2 = n, min1
} else if n <= min2 {
min2 = n
}
if n >= max1 {
max1, max2, max3 = n, max1, max2
} else if n >= max2 {
max2, max3 = n, max2
} else if n >= max3 {
max3 = n
}
}
p1 := min1 * min2 * max1
p2 := max3 * max2 * max1
if p1 > p2 {
return p1
}
return p2
}
<file_sep>/codility/05-prefix-sums/genomic-range-query/genomic.go
package genomic
import (
"strings"
)
// O(N + M)
// https://app.codility.com/demo/results/trainingJ65WK3-RK2/
// Detected time complexity: O(N + M)
func Solution1(S string, P []int, Q []int) []int {
var genoms [3][]int
genoms[0] = make([]int, len(S)+1) // A
genoms[1] = make([]int, len(S)+1) // C
genoms[2] = make([]int, len(S)+1) // G
for i, v := range S {
a, c, g := 0, 0, 0
switch v {
case 'A':
a = 1
case 'C':
c = 1
case 'G':
g = 1
}
genoms[0][i+1] = genoms[0][i] + a
genoms[1][i+1] = genoms[1][i] + c
genoms[2][i+1] = genoms[2][i] + g
}
result := make([]int, len(P))
for i := 0; i < len(P); i++ {
fromIndex := P[i]
toIndex := Q[i] + 1
if genoms[0][toIndex]-genoms[0][fromIndex] > 0 {
result[i] = 1
} else if genoms[1][toIndex]-genoms[1][fromIndex] > 0 {
result[i] = 2
} else if genoms[2][toIndex]-genoms[2][fromIndex] > 0 {
result[i] = 3
} else {
result[i] = 4
}
}
return result
}
// Failed: extreme_large (all max ranges)
// https://app.codility.com/demo/results/training73WDJH-RUG/
// Detected time complexity: O(N + M)
func Solution(S string, P []int, Q []int) []int {
result := make([]int, len(P))
for i := 0; i < len(P); i++ {
strip := S[P[i] : Q[i]+1]
if strings.Contains(strip, "A") {
result[i] = 1
} else if strings.Contains(strip, "C") {
result[i] = 2
} else if strings.Contains(strip, "G") {
result[i] = 3
} else {
result[i] = 4
}
}
return result
}
<file_sep>/crypto/ecdh/ecdh.go
package ecdh
import (
"crypto"
"encoding/hex"
"errors"
"io"
)
var (
ErrInvalidKey = errors.New("pointer to [32]byte key is required")
ErrInvalidData = errors.New("[32]byte data is required")
)
type ECDH interface {
GenerateKeyPair(rand io.Reader) (crypto.PrivateKey, crypto.PublicKey, error)
ComputeSharedSecret(private crypto.PrivateKey, peerPublic crypto.PublicKey) []byte
}
var Curve25519 ECDH
func init() {
Curve25519 = ecdhCurve25519{}
}
func PublicKeyToHexString(public crypto.PublicKey) (string, error) {
switch buf := public.(type) {
case *[32]byte:
return hex.EncodeToString(buf[:]), nil
default:
return "", ErrInvalidKey
}
}
func PublicKeyFromHexString(data string) (crypto.PublicKey, error) {
var public [32]byte
buf, err := hex.DecodeString(data)
if err != nil {
return nil, err
}
if len(buf) != 32 {
return nil, ErrInvalidData
}
copy(public[:], buf)
return &public, nil
}
<file_sep>/exercism/luhn/luhn.go
package luhn
import (
"regexp"
"strconv"
"strings"
)
// Valid determines whether or not a number is valid per the Luhn formula.
func Valid(id string) bool {
id = strings.Replace(id, " ", "", -1)
re := regexp.MustCompile(`^\d{2,}$`)
if !re.MatchString(id) {
return false
}
arr := strings.Split(id, "")
sum := 0
alternate := false
for i := len(arr) - 1; i >= 0; i-- {
n, _ := strconv.Atoi(arr[i])
// if err != nil {
// return false
// }
if alternate {
n <<= 1
if n > 9 {
n -= 9
}
}
alternate = !alternate
sum += n
}
return (sum%10 == 0)
}
<file_sep>/patterns/concurrency/sieve.go
// A concurrent prime sieve
package main
import "fmt"
// Send the sequence 2, 3, 4, ... to channel 'ch'.
func Generate(ch chan<- int) {
for i := 2; ; i++ {
fmt.Print("i=", i)
ch <- i // Send 'i' to channel 'ch'.
}
}
// Copy the values from channel 'in' to channel 'out',
// removing those divisible by 'prime'.
func Filter(in <-chan int, out chan<- int, prime int) {
for {
i := <-in // Receive value from 'in'.
if i%prime != 0 {
out <- i // Send 'i' to 'out'.
}
}
}
// The prime sieve: Daisy-chain Filter processes.
func main() {
ch := make(chan int) // Create a new channel.
go Generate(ch) // Launch Generate goroutine.
for i := 0; i < 10; i++ {
prime := <-ch
fmt.Println("prime=", prime)
ch1 := make(chan int)
go Filter(ch, ch1, prime)
ch = ch1
}
}
/*
i=2 i=3
prime= 2
prime= 3
i=4 i=5 i=6 i=7 i=8 i=9 i=10
prime= 5
prime= 7
i=11 i=12 i=13 i=14 i=15 i=16 i=17 i=18 i=19 i=20 i=21 i=22
prime= 11
prime= 13
prime= 17
i=23 i=24 i=25 i=26 i=27 i=28 i=29 i=30 i=31 i=32 i=33 i=34 i=35 i=36 i=37 i=38 i=39 i=40 i=41 i=42 i=43 i=44 i=45 i=46
prime= 19
prime= 23
prime= 29
i=47
*/<file_sep>/codility/05-prefix-sums/passing-cars/passing_cars_test.go
package passingcars
import (
"testing"
)
func TestPassingCars(t *testing.T) {
for _, c := range testCases {
output := PassingCars(c.input)
if output != c.expected {
t.Fatalf("FAIL: %v\nInput: %v\nexpected: %v\ngot: %v", c.description, c.input, c.expected, output)
}
t.Logf("PASS: %v", c.input)
}
}
func BenchmarkPassingCars(b *testing.B) {
for i := 0; i < b.N; i++ {
for _, c := range testCases {
PassingCars(c.input)
}
}
}
var testCases = []struct {
description string
input []int
expected int
}{
{
description: "five pairs of passing cars",
input: []int{0, 1, 0, 1, 1},
expected: 5,
},
{
description: "five pairs of passing cars",
input: []int{0, 1, 0, 1, 1, 0},
expected: 5,
},
{
description: "seven pairs of passing cars",
input: []int{0, 1, 0, 1, 1, 1},
expected: 7,
},
{
description: "eight pairs of passing cars",
input: []int{0, 1, 0, 1, 1, 0, 1},
expected: 8,
},
{
description: "zero pairs of passing cars",
input: []int{1, 1, 1},
expected: 0,
},
{
description: "zero pairs of passing cars",
input: []int{0, 0, 0},
expected: 0,
},
}
<file_sep>/avatarme/main.go
package main
import (
"log"
"os"
"image/png"
"./identicon"
)
func main() {
text := "<EMAIL>"
icon, err := identicon.Identicon(text)
if err != nil {
log.Fatal(err)
}
file, err := os.Create("identicon.png")
if err != nil {
log.Fatalf("Fail to create identicon.png: %v", err)
}
if err := png.Encode(file, icon); err != nil {
file.Close()
log.Fatal(err)
}
if err := file.Close(); err != nil {
log.Fatal(err)
}
log.Println("Identicon successfully created.")
}
<file_sep>/codility/04-counting-elements/frog-river-one/frog_river_one.go
package main
import (
"fmt"
)
func Solution(X int, A []int) int {
expectedTotal := X * (X + 1) / 2
total := 0
seen := make(map[int]struct{})
for i, v := range A {
if _, ok := seen[v]; !ok {
seen[v] = struct{}{}
total += v
}
if total == expectedTotal {
return i
}
}
return -1
}
func main() {
fmt.Println(Solution(5, []int{1, 3, 1, 4, 2, 3, 5, 4})) // expected 6
fmt.Println(Solution(3, []int{1, 3, 1, 3, 2, 1, 3})) // expected 4
}
// https://app.codility.com/programmers/lessons/4-counting_elements/frog_river_one/
// https://app.codility.com/demo/results/trainingC7Q4JH-QU6/
// Detected time complexity:
// O(N)<file_sep>/exercism/gigasecond/gigasecond.go
// Package gigasecond provides functions that calculates the moment when someone has lived for 10^9 seconds.
package gigasecond
import (
"time"
)
// AddGigasecond calculates the moment when someone has lived for 10^9 seconds.
func AddGigasecond(t time.Time) time.Time {
return t.Add(1e9 * time.Second)
}
<file_sep>/codility/others/phone-number-format/phone_format.go
package main
import (
"fmt"
"strings"
)
const (
space = " "
dash = "-"
)
func format(phone string) string {
phone = removeNonDigits(phone)
if len(phone) <= 3 {
return phone
}
var result strings.Builder
i := 0
for i < len(phone)-4 {
result.WriteString(phone[i : i+3])
result.WriteString(dash)
i += 3
}
remainingDigits := len(phone) - i
switch remainingDigits {
case 2, 3:
result.WriteString(phone[i:])
break
case 4:
result.WriteString(phone[i : i+2])
result.WriteString(dash)
result.WriteString(phone[i+2:])
break
}
return result.String()
}
func removeNonDigits(s string) string {
s = strings.ReplaceAll(s, space, "")
s = strings.ReplaceAll(s, dash, "")
return s
}
func main() {
fmt.Println(format("")) //
fmt.Println(format("-")) //
fmt.Println(format("0-")) // 0
fmt.Println(format("00")) // 00
fmt.Println(format("0-0")) // 00
fmt.Println(format("00-")) // 00
fmt.Println(format("014-")) // 014
fmt.Println(format("01-4-")) // 014
fmt.Println(format("01-4-1")) // 01-41
fmt.Println(format("00-44 48 5555 8361")) // 004-448-555-583-61
fmt.Println(format("0 - 22 1985-324")) // 022-198-53-24
fmt.Println(format("555372654")) // 555-372-654
}
<file_sep>/avatarme/identicon/identicon.go
package identicon
import (
"errors"
"crypto/sha1"
"image"
"image/color"
"image/draw"
)
const (
IconWidth = 250
CellWidth = IconWidth / 5
)
func Identicon(data string) (image.Image, error) {
if len(data) < 15 {
return nil, errors.New("A text of at least 15 characters is required.")
}
// hashing the input data
input := []byte(data)
hash := sha1.Sum(input)
// background is grey by default
background := color.RGBA{240, 240, 240, 255}
// the last three of the hash is used to generate the foreground color
r := hash[17]
g := hash[18]
b := hash[19]
foreground := color.RGBA{r, g, b, 255}
icon := image.NewRGBA(image.Rect(0, 0, IconWidth, IconWidth))
var p0, p1 image.Point
// the first 15th of the hash are used to turn pixels on or off depending on even or odd values.
for i := 0; i < 15; i++ {
c := &image.Uniform{background}
if hash[i]%2 == 0 {
c = &image.Uniform{foreground}
}
// 5 columns
if i < 5 { // 3th column is drawn first
p0 = image.Pt(2*CellWidth, i*CellWidth)
p1 = image.Pt(3*CellWidth, (i+1)*CellWidth)
drawBlock(icon, p0, p1, c)
} else if i < 10 { // then 2nd and 4th
p0 = image.Pt(1*CellWidth, (i-5)*CellWidth)
p1 = image.Pt(2*CellWidth, (i-4)*CellWidth)
drawBlock(icon, p0, p1, c)
p0 = image.Pt(3*CellWidth, (i-5)*CellWidth)
p1 = image.Pt(4*CellWidth, (i-4)*CellWidth)
drawBlock(icon, p0, p1, c)
} else if i < 15 { // lastly, first and fifth
p0 = image.Pt(0*CellWidth, (i-10)*CellWidth)
p1 = image.Pt(1*CellWidth, (i-9)*CellWidth)
drawBlock(icon, p0, p1, c)
p0 = image.Pt(4*CellWidth, (i-10)*CellWidth)
p1 = image.Pt(5*CellWidth, (i-9)*CellWidth)
drawBlock(icon, p0, p1, c)
}
}
return icon, nil
}
func drawBlock(dst draw.Image, p0 image.Point, p1 image.Point, src image.Image) {
draw.Draw(dst, image.Rectangle{p0, p1}, src, image.ZP, draw.Src)
}
<file_sep>/exercism/sieve/sieve.go
package sieve
// Sieve finds all the primes from 2 up to a given number using Sieve of Eratosthenes.
func Sieve(limit int) (primes []int) {
if limit <= 1 {
return
}
marker := make([]bool, limit+1)
for p := 2; p*p <= limit; p++ {
if !marker[p] {
for i := p * p; i <= limit; i += p {
marker[i] = true
}
}
}
for i := 2; i <= limit; i++ {
if !marker[i] {
primes = append(primes, i)
}
}
return
}
<file_sep>/codility/09-max-slice-problem/max-double-slice-sum/max_double_slice_sum.go
package main
import (
"fmt"
)
// https://app.codility.com/demo/results/trainingRJR4TK-YDV/
// Detected time complexity: O(N)
func max(A []int) int {
forwardSum := make([]int, len(A))
backwardSum := make([]int, len(A))
for i := 1; i < len(A)-1; i++ {
if forwardSum[i-1]+A[i] > 0 {
forwardSum[i] = forwardSum[i-1] + A[i]
} else {
forwardSum[i] = 0
}
}
for i := len(A) - 2; i >= 1; i-- {
if backwardSum[i+1]+A[i] > 0 {
backwardSum[i] = backwardSum[i+1] + A[i]
} else {
backwardSum[i] = 0
}
}
max := 0
for i := 0; i < len(A)-2; i++ {
if forwardSum[i]+backwardSum[i+2] > max {
max = forwardSum[i] + backwardSum[i+2]
}
}
return max
}
func main() {
fmt.Println(max([]int{3, 2, 6, -1, 4, 5, -1, 2}))
// forwardSum: [0, 2, 8, 7, 11, 16, 15, 0]
// backwardSum: [0, 16, 14, 8, 9, 5, 0, 0]
// result: 17
}
<file_sep>/tour/concurrency/20-Pi-no-routine.go
package main
import (
"fmt"
"math"
)
func main() {
pi := 0.0
for i := 0; i <= 5000; i++ {
pi += 4 * math.Pow(-1, float64(i)) / (2*float64(i) + 1)
}
fmt.Println(pi)
}
<file_sep>/codility/10-prime-composite-numbers/count-factors/count_factors.go
package main
import (
"fmt"
)
// https://app.codility.com/demo/results/training8E34M3-HNE/
// Detected time complexity: O(sqrt(N))
func count(N int) int {
c := 0
i := 1
for i*i < N {
if N%i == 0 {
c += 2
}
i++
}
if i*i == N {
c++
}
return c
}
func main() {
fmt.Println(count(24)) // 8
}
<file_sep>/tour/basic/05-if.go
package main
import (
"fmt"
"math"
)
func sqrt(x float64) string {
if x < 0 {
return sqrt(-x) + "i"
}
return fmt.Sprint(math.Sqrt(x))
}
func main() {
fmt.Println(sqrt(4))
fmt.Println(sqrt(-4))
fmt.Println(
pow(3, 2, 10),
pow(3, 3, 20),
)
}
func pow(x, n, lim float64) float64 {
// If with a short statement
// Variables declared by the statement are
// - only in scope until the end of the if.
// - also available inside any of the else blocks
if v := math.Pow(x, n); v < lim {
return v
} else {
fmt.Printf("%g >= %g\n", v, lim)
}
// can't use v here, though
return lim
}
<file_sep>/codility/04-counting-elements/missing-integer/missing_integer.go
package missing
import (
"sort"
)
// O(n*log(n))
// https://app.codility.com/demo/results/trainingPYGD47-NCS/
// Detected time complexity: O(N) or O(N * log(N))
func Solution1(A []int) int {
sort.Ints(A)
min := 1
for _, v := range A {
if v == min {
min++
}
}
return min
}
// O(n)
// https://app.codility.com/demo/results/trainingT3JKNV-YMX/
// Detected time complexity: O(N) or O(N * log(N))
func Solution(A []int) int {
positiveElems := make(map[int]struct{})
for _, v := range A {
if v > 0 {
positiveElems[v] = struct{}{}
}
}
min := 1
for {
if _, prs := positiveElems[min]; !prs {
return min
}
min++
}
}
<file_sep>/codility/05-prefix-sums/count-div/count_div_test.go
package div
import (
"testing"
)
func TestSolution(t *testing.T) {
for _, c := range testCases {
output := Solution(c.input[0], c.input[1], c.input[2])
if output != c.expected {
t.Fatalf("\nFAIL: %v\nInput: [%v..%v] and %v\nexpected: %v\ngot: %v", c.description, c.input[0], c.input[1], c.input[2], c.expected, output)
}
t.Logf("PASS: %v", c.description)
}
}
func BenchmarkSolution(b *testing.B) {
for i := 0; i < b.N; i++ {
for _, c := range testCases {
Solution(c.input[0], c.input[1], c.input[2])
}
}
}
var testCases = []struct {
description string
input []int
expected int
}{
{
description: "No.1",
input: []int{6, 11, 2},
expected: 3,
},
{
description: "No.2",
input: []int{11, 14, 2},
expected: 2,
},
{
description: "No.3",
input: []int{11, 345, 17},
expected: 20,
},
{
description: "No.4",
input: []int{0, 1, 11},
expected: 1,
},
{
description: "No.5",
input: []int{10, 10, 5},
expected: 1,
},
{
description: "No.6",
input: []int{10, 10, 7},
expected: 0,
},
{
description: "No.7",
input: []int{10, 10, 20},
expected: 0,
},
}
<file_sep>/codility/02-arrays/odd-occurrences/odd.go
package main
import (
"fmt"
)
func Solution(A []int) int {
m := make(map[int]int)
for _, v := range A {
m[v]++
}
for k := range m {
if m[k]%2 != 0 {
return k
}
}
return -1
}
func main() {
fmt.Println(Solution([]int{9, 3, 9, 3, 9, 7, 9}))
}
// https://app.codility.com/programmers/lessons/2-arrays/odd_occurrences_in_array/<file_sep>/exercism/isbn-verifier/isbn_verifier.go
package isbn
import (
"strconv"
"strings"
)
// IsValidISBN validates the input ISBN number.
func IsValidISBN(isbn string) bool {
isbn = strings.Replace(isbn, "-", "", -1)
if len(isbn) != 10 {
return false
}
arr := strings.Split(isbn, "")
sum := 0
if arr[len(arr)-1] == "X" {
sum += 10
arr = arr[0 : len(arr)-1]
}
for i := 0; i < len(arr); i++ {
v, err := strconv.Atoi(arr[i])
if err != nil {
return false
}
sum += v * (10 - i)
}
if sum%11 == 0 {
return true
}
return false
}
<file_sep>/tour/image-api/two-colorful-rectangles.go
package main
import (
"image"
"image/color"
"image/draw"
"image/png"
"os"
)
func main() {
new_png_file := "two_rectangles.png"
myimage := image.NewRGBA(image.Rect(0, 0, 220, 220)) // x1,y1, x2,y2
mygreen := color.RGBA{0, 100, 0, 255} // R, G, B, Alpha
// backfill entire surface with green
draw.Draw(myimage, myimage.Bounds(), &image.Uniform{mygreen}, image.ZP, draw.Src)
red_rect := image.Rect(60, 80, 120, 160) // geometry of 2nd rectangle
myred := color.RGBA{200, 0, 0, 255}
// create a red rectangle atop the green surface
draw.Draw(myimage, red_rect, &image.Uniform{myred}, image.ZP, draw.Src)
myfile, _ := os.Create(new_png_file) // ... now lets save imag
png.Encode(myfile, myimage)
}
<file_sep>/codility/07-stacks-queues/nesting/nesting.go
package main
import (
"fmt"
)
// Performance issue:
// https://app.codility.com/demo/results/trainingD69EKZ-5BP/
// Detected time complexity: O(N)
// https://app.codility.com/demo/results/trainingZEWMVA-SUN/
// Detected time complexity: O(N)
func Solution(S string) int {
if 0 == len(S) {
return 1
}
if 0 != len(S)%2 {
return 0
}
stack := []rune{}
for _, r := range S {
switch r {
case '(':
stack = append(stack, r)
case ')':
len := len(stack)
if 0 == len {
return 0
}
if stack[len-1] != '(' {
return 0
}
stack = stack[:len-1]
default:
return 0
}
}
if 0 == len(stack) {
return 1
}
return 0
}
func main() {
fmt.Println(Solution("")) // 1
fmt.Println(Solution("(")) // 0
fmt.Println(Solution("(()(())())")) // 1
fmt.Println(Solution("())")) // 0
}
<file_sep>/codility/11-sieve-of-eratoshenes/sieve.go
package main
import (
"fmt"
)
func primes(n int) []int {
marker := make([]bool, n+1)
for p := 2; p*p <= n; p++ {
if !marker[p] {
for i := p * p; i <= n; i += p {
marker[i] = true
}
}
}
prime := []int{}
for i := 2; i <= n; i++ {
if !marker[i] {
prime = append(prime, i)
}
}
return prime
}
func factors(n int64) []int64 {
r := make([]int64, 0)
for i := int64(2); i <= n; i++ {
for n%i == 0 {
r = append(r, i)
n /= i
}
}
return r
}
func main() {
fmt.Println(primes(10)) // 2, 3, 5, 7
fmt.Println(primes(17)) /// 2, 3, 5, 7, 11, 13, 17
fmt.Println(primes(30)) // 2, 3, 5, 7, 11, 13, 17, 19, 23, 29
}
<file_sep>/exercism/grains/grains.go
// Package grains provides function that calculates the number of grains of wheat on a chessboard given that the number on each square doubles.
package grains
import "errors"
// Square returns the number of grains on a square.
func Square(square int) (uint64, error) {
if square < 1 || square > 64 {
return 0, errors.New("square must be from 1 to 64")
}
return 1 << uint64(square-1), nil
}
// Total returns the total number of grains there could be on a chessboard.
func Total() uint64 {
return 1<<64 - 1
}
<file_sep>/codility/05-prefix-sums/passing-cars/passing_cars.go
package passingcars
const max = 1000000000
// https://app.codility.com/demo/results/trainingVWFGAN-ZNG/
// Detected time complexity:
// O(N)
func PassingCars1(A []int) int {
total := 0
count := 0
for i := 0; i < len(A); i++ {
if A[i] == 0 {
count++
} else {
total += count
}
if total > max {
return -1
}
}
return total
}
// O(n)
// https://app.codility.com/demo/results/training8HTB7M-TV9/
// Detected time complexity: O(N)
func PassingCars(A []int) int {
total := 0
for _, v := range A {
total += v
}
passingCars := 0
leftTotal := 0
for _, v := range A {
if v == 0 {
passingCars += total - leftTotal
if passingCars > max {
return -1
}
}
leftTotal += v
}
return passingCars
}
<file_sep>/exercism/parallel-letter-frequency/frequency.go
package letter
import (
"sync"
)
// FreqMap records the frequency of each rune in a given text.
type FreqMap map[rune]int
// Frequency counts the frequency of each rune in a given text and returns this
// data as a FreqMap.
func Frequency(s string) FreqMap {
m := FreqMap{}
for _, r := range s {
m[r]++
}
return m
}
// ConcurrentFrequency is the concurrent version of Frequency.
func ConcurrentFrequency(strs []string) FreqMap {
var counter = struct {
sync.Mutex
freq FreqMap
}{freq: map[rune]int{}}
var wg sync.WaitGroup
wg.Add(len(strs))
for _, s := range strs {
go func(s string) {
for _, r := range s {
counter.Lock()
counter.freq[r]++
counter.Unlock()
}
wg.Done()
}(s)
}
wg.Wait()
return counter.freq
}
<file_sep>/codility/04-counting-elements/max-counters/max_counters.go
package main
import (
"fmt"
)
func Solution(N int, A []int) []int {
counter := make([]int, N)
max := 0
lastMax := 0
for _, v := range A {
if v >= 1 && v <= N {
cv := counter[v-1]
if cv < lastMax {
cv = lastMax
}
cv++
if cv > max {
max = cv
}
counter[v-1] = cv
} else if v == N+1 {
lastMax = max
}
}
for i, v := range counter {
if v < lastMax {
counter[i] = lastMax
}
}
return counter
}
func main() {
fmt.Println(Solution(5, []int{3, 4, 4, 6, 1, 4, 4})) // expected: [3, 2, 2, 4, 2]
}
// https://app.codility.com/programmers/lessons/4-counting_elements/max_counters/
// https://app.codility.com/demo/results/trainingAWNA36-FCR/
// Detected time complexity:
// O(N + M)
<file_sep>/exercism/space-age/space_age.go
// Package space provides function to calculate Earth-years old.
package space
// Solar system planets
const (
Earth = "Earth"
Mercury = "Mercury"
Venus = "Venus"
Mars = "Mars"
Jupiter = "Jupiter"
Saturn = "Saturn"
Uranus = "Uranus"
Neptune = "Neptune"
)
// Planet orbital periods
const (
EarthObPeriod float64 = 31557600
MercuryObPeriod float64 = EarthObPeriod * 0.2408467
VenusObPeriod float64 = EarthObPeriod * 0.61519726
MarsObPeriod float64 = EarthObPeriod * 1.8808158
JupiterObPeriod float64 = EarthObPeriod * 11.862615
SaturnObPeriod float64 = EarthObPeriod * 29.447498
UranusObPeriod float64 = EarthObPeriod * 84.016846
NeptuneObPeriod float64 = EarthObPeriod * 164.79132
)
// Age calculates and returns Earth-years old.
func Age(seconds float64, planet string) float64 {
switch planet {
case Earth:
return seconds / EarthObPeriod
case Mercury:
return seconds / MercuryObPeriod
case Venus:
return seconds / VenusObPeriod
case Mars:
return seconds / MarsObPeriod
case Jupiter:
return seconds / JupiterObPeriod
case Saturn:
return seconds / SaturnObPeriod
case Uranus:
return seconds / UranusObPeriod
case Neptune:
return seconds / NeptuneObPeriod
}
return 0.00
}
<file_sep>/exercism/paasio/paasio.go
package paasio
import (
"io"
"sync"
)
type counter struct {
mux *sync.Mutex
nbytes int64
nops int
}
func newCounter() counter {
return counter{mux: new(sync.Mutex)}
}
func (c *counter) count(nbytes int) {
c.mux.Lock()
defer c.mux.Unlock()
c.nops++
c.nbytes += int64(nbytes)
}
func (c *counter) stats() (nbytes int64, nops int) {
c.mux.Lock()
defer c.mux.Unlock()
return c.nbytes, c.nops
}
type readCounter struct {
r io.Reader
counter
}
func (rc *readCounter) Read(p []byte) (n int, err error) {
n, err = rc.r.Read(p)
rc.count(n)
return
}
func (rc *readCounter) ReadCount() (nbytes int64, nops int) {
return rc.stats()
}
type writeCounter struct {
w io.Writer
counter
}
func (wc *writeCounter) Write(p []byte) (n int, err error) {
n, err = wc.w.Write(p)
wc.count(n)
return
}
func (wc *writeCounter) WriteCount() (nbytes int64, nops int) {
return wc.stats()
}
type readWriteCounter struct {
ReadCounter
WriteCounter
}
// NewReadCounter returns an implementation of ReadCounter. Calls to
// r.Read() are not guaranteed to be synchronized.
func NewReadCounter(r io.Reader) ReadCounter {
return &readCounter{r: r, counter: newCounter()}
}
// NewWriteCounter returns an implementation of WriteCounter. Calls to
// w.Write() are not guaranteed to be synchronized.
func NewWriteCounter(w io.Writer) WriteCounter {
return &writeCounter{w: w, counter: newCounter()}
}
// NewReadWriteCounter returns an implementation of ReadWriteCounter.
// Calls to rw.Write() and rw.Read() are not guaranteed to be synchronized.
func NewReadWriteCounter(rw io.ReadWriter) ReadWriteCounter {
return &readWriteCounter{NewReadCounter(rw), NewWriteCounter(rw)}
}
<file_sep>/tour/basic/12-function-closure-easier-to-understand.go
package main
import (
"fmt"
)
func main() {
f := inc()
fmt.Println(f())
fmt.Println(f())
fmt.Println(f())
fmt.Println(f())
fmt.Println(f())
fmt.Println("New...")
g := inc()
fmt.Println(g())
fmt.Println(g())
fmt.Println(g())
fmt.Println(g())
fmt.Println(g())
}
func inc() func() int {
x := 0
return func() int {
x++
return x
}
}
/*
1
2
3
4
5
New...
1
2
3
4
5
*/
<file_sep>/exercism/rna-transcription/rna_transcription.go
// Package strand provides function to transcibe dna to rna
package strand
import (
"bytes"
)
// ToRNA transcibes dna to rna
func ToRNA(dna string) string {
if dna == "" {
return ""
}
var buf bytes.Buffer
for _, nucleotide := range dna {
switch nucleotide {
case 'G':
buf.WriteRune('C')
case 'C':
buf.WriteRune('G')
case 'T':
buf.WriteRune('A')
case 'A':
buf.WriteRune('U')
}
}
return buf.String()
}
<file_sep>/codility/others/string-obtained-string/obtained.go
package main
import (
"fmt"
"strings"
)
const (
nothing = `NOTHING`
add = `ADD %s`
change = `CHANGE %s %s`
move = `MOVE %s`
impossible = `IMPOSSIBLE`
)
func equal(S, T string) string {
if S == T {
return nothing
}
return ""
}
func addable(S, T string) string {
if len(S) == len(T)-1 && S == T[:len(T)-1] {
return fmt.Sprintf(add, T[len(T)-1:])
}
return ""
}
func changable(S, T string) string {
n := len(S)
m := len(T)
if n == m {
diffIndexes := []int{}
i := 0
for i < n {
if S[i] != T[i] {
diffIndexes = append(diffIndexes, i)
if len(diffIndexes) > 1 {
return ""
}
}
i++
}
return fmt.Sprintf(change, string(S[diffIndexes[0]]), string(T[diffIndexes[0]]))
}
return ""
}
func moveable(S, T string) string {
n := len(S)
m := len(T)
if n == m {
for i := 0; i < n; i++ {
if S[i] != T[i] {
s := S[i+1:]
t := strings.Replace(T[i:], string(S[i]), "", 1)
if s == t {
return fmt.Sprintf(move, string(S[i]))
}
return ""
}
}
}
return ""
}
func obtain(S, T string) string {
S = strings.ToLower(S)
T = strings.ToLower(T)
result := equal(S, T)
if result != "" {
return result
}
result = addable(S, T)
if result != "" {
return result
}
result = changable(S, T)
if result != "" {
return result
}
result = moveable(S, T)
if result != "" {
return result
}
return impossible
}
func main() {
fmt.Println(obtain("", "")) // NOTHING
fmt.Println(obtain("a", "a")) // NOTHING
fmt.Println(obtain("a", "A")) // NOTHING
fmt.Println(obtain("abcd", "AbCD")) // NOTHING
fmt.Println(obtain("0", "odd")) // IMPOSSIBLE
fmt.Println(obtain("nice", "nicer")) // ADD r
fmt.Println(obtain("xx", "xXx")) // ADD x
fmt.Println(obtain("test", "tent")) // CHANGE s n
fmt.Println(obtain("text", "tent")) // CHANGE x n
fmt.Println(obtain("text", "texx")) // CHANGE t x
fmt.Println(obtain("test", "tenk")) // IMPOSSIBLE
fmt.Println(obtain("beans", "banes")) // MOVE e
fmt.Println(obtain("beanse", "banese")) // MOVE e
}
<file_sep>/codility/08-leader/equi-leader/equi_leader.go
package main
import (
"fmt"
)
// https://app.codility.com/demo/results/training78FUEM-78H/
// O(N)
func Solution(A []int) int {
if 0 == len(A) {
return 0
}
value := A[0]
size := 0
for _, a := range A {
if 0 == size {
size++
value = a
} else {
if a != value {
size--
} else {
size++
}
}
}
candidate := -1
if 0 != size {
candidate = value
}
count := 0
for _, a := range A {
if a == candidate {
count++
}
}
leader := -1
if count > len(A)/2 {
leader = candidate
} else {
return 0
}
equi := 0
left := 0
right := 0
for i, a := range A {
if a == leader {
left++
right = count - left
}
if (left > (i+1)/2) && (right > (len(A)-(i+1))/2) {
equi++
}
}
return equi
}
func main() {
fmt.Println(Solution([]int{4, 3, 4, 4, 4, 2})) // 2
}
<file_sep>/codility/05-prefix-sums/min-avg/min_avg_test.go
package minavg
import (
"testing"
)
func TestSolution(t *testing.T) {
for _, c := range testCases {
output := Solution(c.input)
if output != c.expected {
t.Fatalf("\nFAIL: %v\nInput: %v\nexpected: %v\ngot: %v", c.description, c.input, c.expected, output)
}
t.Logf("PASS: %v", c.description)
}
}
func BenchmarkSolution(b *testing.B) {
for i := 0; i < b.N; i++ {
for _, c := range testCases {
Solution(c.input)
}
}
}
var testCases = []struct {
description string
input []int
expected int
}{
{
description: "Positive integers",
input: []int{4, 2, 2, 5, 1, 5, 8},
expected: 1,
},
{
description: "Nagative integers",
input: []int{-3, -5, -8, -4, -10},
expected: 2,
},
}
<file_sep>/codility/09-max-slice-problem/max-profit/max_profit.go
package main
import (
"fmt"
)
// O(n)
// https://app.codility.com/demo/results/trainingUZ5J8F-4S6/
func max(A []int) int {
minDailyPrice := 200000
maxProfit := 0
for _, a := range A {
if a < minDailyPrice {
minDailyPrice = a
}
if a-minDailyPrice > maxProfit {
maxProfit = a - minDailyPrice
}
}
return maxProfit
}
func main() {
fmt.Println(max([]int{23171, 21011, 21123, 21366, 21013, 21367})) // 356
}
<file_sep>/codility/04-counting-elements/perm-check/perm_check.go
package main
import (
"fmt"
)
func Solution(A []int) int {
counter := make(map[int]struct{})
for _, v := range A {
if v > len(A) {
return 0
}
counter[v] = struct{}{}
}
if len(counter) == len(A) {
return 1
}
return 0
}
func main() {
fmt.Println(Solution([]int{4, 1, 3, 2})) // 1
fmt.Println(Solution([]int{4, 1, 3})) // 0
}
// https://app.codility.com/demo/results/training9WRW9M-F43/
// Detected time complexity:
// O(N) or O(N * log(N))<file_sep>/codility/04-counting-elements/missing-integer/missing_integer_test.go
package missing
import (
"testing"
)
var testCases = []struct {
description string
input []int
expected int
}{
{
description: "positve with duplication",
input: []int{1, 3, 6, 4, 1, 2},
expected: 5,
},
{
description: "positve incresing integers starting from 1",
input: []int{1, 2, 3},
expected: 4,
},
{
description: "negative only",
input: []int{-1, -3},
expected: 1,
},
{
description: "positve and negative",
input: []int{-1, -3, 0, 4, 2},
expected: 1,
},
{
description: "minimal and maximal values",
input: []int{-1000000, 1000000},
expected: 1,
},
{
description: "a single element",
input: []int{1},
expected: 2,
},
{
description: "a single element",
input: []int{3},
expected: 1,
},
{
description: "a single element",
input: []int{-2},
expected: 1,
},
}
func TestSolution(t *testing.T) {
for _, c := range testCases {
output := Solution(c.input)
if output != c.expected {
t.Fatalf("FAIL: %v\nInput: %v\nexpected: %v\ngot: %v", c.description, c.input, c.expected, output)
}
t.Logf("PASS: %v", c.input)
}
}
func BenchmarkSolution(b *testing.B) {
for i := 0; i < b.N; i++ {
for _, c := range testCases {
Solution(c.input)
}
}
}
<file_sep>/codility/03-time-complexity/frog-jmp/frog_jmp.go
package main
import (
"fmt"
)
func Solution(X int, Y int, D int) int {
dis := Y - X
steps := dis / D
if dis%D == 0 {
return steps
}
return steps + 1
}
func main() {
fmt.Println(Solution(10, 85, 30))
}
// https://app.codility.com/programmers/lessons/3-time_complexity/frog_jmp/
// https://app.codility.com/demo/results/trainingMJ2TT6-F38/
// Detected time complexity:
// O(1)<file_sep>/exercism/alphametics/alphamatics.go
// Package alphametics sfs.
package alphametics
// Solve attempts to solve the alphametics puzzle and return a map of all the letter substitutions for both the puzzle and the addition solution.
func Solve(puzzle string) (map[string]int, error) {
}
<file_sep>/tour/methods-interfaces/04-pointer-receivers.go
package main
import (
"fmt"
"math"
)
type Vertex struct {
X, Y float64
}
func (v Vertex) Abs() float64 {
return math.Sqrt(v.X*v.X + v.Y*v.Y)
}
func (v *Vertex) Scale(f float64) {
v.X = v.X * f
v.Y = v.Y * f
}
func main() {
v := Vertex{3, 4}
v.Scale(10)
// even though v is a value and not a pointer, the method with the pointer receiver is called automatically.
// That is, as a convenience, Go interprets the statement v.Scale(10) as (&v).Scale(10) since the Scale method has a pointer receiver.
fmt.Println(v.Abs())
}
// You can declare methods with pointer receivers.
// Methods with pointer receivers can modify the value to which the receiver points
// Since methods often need to modify their receiver, pointer receivers are more common than value receivers.
<file_sep>/codility/07-stacks-queues/stone-wall/stone_wall.go
package main
import (
"fmt"
)
// https://app.codility.com/demo/results/trainingR3MP65-A8J/
// Detected time complexity: O(N)
func Solution(H []int) int {
stones := 0
stack := []int{}
for _, h := range H {
for 0 != len(stack) && stack[len(stack)-1] > h {
stack = stack[:len(stack)-1]
}
if 0 != len(stack) && stack[len(stack)-1] == h {
continue
} else {
stack = append(stack, h)
stones++
}
}
return stones
}
func main() {
fmt.Println(Solution([]int{8, 8, 5, 7, 9, 8, 7, 4, 8})) // 7
fmt.Println(Solution([]int{3, 2, 1})) // 3
}
<file_sep>/exercism/robot-name/robot_name.go
package robotname
import (
"errors"
"fmt"
"math/rand"
"time"
)
const max = 26 * 26 * 10 * 10 * 10
var counter = 0
var nameDB = map[string]bool{}
func makeName() string {
src := rand.NewSource(time.Now().UnixNano())
rnd := rand.New(src)
c1 := rune('A' + rnd.Int()%26)
c2 := rune('A' + rnd.Int()%26)
n := rnd.Int() % 1000
return fmt.Sprintf("%c%c%03d", c1, c2, n)
}
// Robot has a name.
type Robot struct {
name string
}
// Name returns robot name.
func (r *Robot) Name() (string, error) {
if r.name == "" {
if err := r.Reset(); err != nil {
return "", err
}
}
return r.name, nil
}
// Reset resets robot name.
func (r *Robot) Reset() error {
if counter > max {
return errors.New("namespace is exhausted")
}
name := makeName()
for nameDB[name] {
name = makeName()
}
nameDB[name] = true
r.name = name
counter++
return nil
}
<file_sep>/tour/others/const-iota.go
package main
import (
"fmt"
)
const (
Sunday = iota
Monday
Tuesday
Wednesday
Thursday
Friday
Partyday
sfdsds
numbdderOfDays // this constant is not exported
)
func main() {
fmt.Println("Hello, playground")
fmt.Println(Sunday)
fmt.Println(Wednesday)
fmt.Println(Partyday)
fmt.Println(numbdderOfDays)
}
<file_sep>/codility/01-iterations/binary_gap.go
package main
import (
"fmt"
"strconv"
"strings"
)
func Solution(N int) int {
str := strconv.FormatInt(int64(N), 2)
str = strings.TrimRight(str, "0")
arr := strings.Split(str, "1")
longest := 0
for _, v := range arr {
if len(v) > longest {
longest = len(v)
}
}
return longest
}
func main() {
fmt.Println(Solution(2147483646))
}
// https://app.codility.com/programmers/lessons/1-iterations/binary_gap/<file_sep>/exercism/armstrong-numbers/armstrong.go
package armstrong
import (
"math"
"strconv"
)
// IsNumber checks whether a number is an Armstrong number,
// that is the sum of its own digits each raised to the power of the number of digits.
func IsNumber(n int) bool {
return isNumberVb(n)
}
func isNumberVa(n int) bool {
sum := 0
s := strconv.Itoa(n)
y := float64(len(s))
for _, v := range s {
x, _ := strconv.Atoi(string(v))
sum += int(math.Pow(float64(x), y))
}
if sum == n {
return true
}
return false
}
func isNumberVb(n int) bool {
y := float64(int(math.Log10(float64(n))) + 1)
sum := 0
i := n
for {
x := float64(i % 10)
sum += int(math.Pow(x, y))
i /= 10
if i == 0 {
break
}
}
if sum == n {
return true
}
return false
}<file_sep>/codility/06-sorting/triangle/trianlge.go
package triangle
import (
"sort"
)
// https://app.codility.com/demo/results/training8VR3Q4-TKG/
// Detected time complexity:
// O(N*log(N))
func Solution(A []int) int {
sort.Ints(A)
for i := 0; i < len(A)-2; i++ {
if A[i]+A[i+1] > A[i+2] {
return 1
}
}
return 0
}
<file_sep>/codility/others/trim/trim.go
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(Solution("The quick brown fox jumps over the lazy dog", 39))
}
func Solution(message string, K int) string {
if len(message) < K {
return message
}
arr := strings.Split(message, " ")
for i := len(arr); i > 1; i-- {
result := strings.Join(arr[:i], " ")
if len(result) <= K {
return result
}
}
return ""
}
<file_sep>/codility/others/nth-highest-sums/main.go
package main
func main() {
}
func findHighestSums(lists [][]int, n int) {
}
<file_sep>/codility/06-sorting/disc-intersections/disc.go
package disc
const limit = 10000000
// O(N) complexity and O(N) memory
// https://app.codility.com/demo/results/training39GYB5-J3P/
// Detected time complexity:
// O(N*log(N)) or O(N)
func Solution(A []int) int {
totalDiscs := len(A)
startPts := make([]int, totalDiscs)
endPts := make([]int, totalDiscs)
for i, a := range A {
start := i - a
if start < 0 {
start = 0
}
end := i + a
if end > (totalDiscs - 1) {
end = totalDiscs - 1
}
startPts[start]++
endPts[end]++
}
result := 0
activeDiscs := 0
for i := 0; i < totalDiscs; i++ {
if startPts[i] > 0 {
result += activeDiscs * startPts[i]
result += startPts[i] * (startPts[i] - 1) / 2
if result > limit {
return -1
}
activeDiscs += startPts[i]
}
activeDiscs -= endPts[i]
}
return result
}
<file_sep>/tour/basic/05-loop.go
package main
import "fmt"
func main() {
// loops
fmt.Println("I'm looping...")
for i := 0; i < 10; i++ {
fmt.Println(i)
}
sum := 0
for i := 0; i <= 100; i++ {
sum += i
}
fmt.Println("sum: ", sum)
// while loop
i := 0
for i < 5 {
fmt.Println(i)
i++
}
// forever
// for {
// }
// loop + array
arrl := []string{"a", "b", "c"}
for index, value := range arrl {
fmt.Println("index: ", index, " - value: ", value)
}
// loop + map
mapl := make(map[string]string)
mapl["a"] = "alpha"
mapl["b"] = "beta"
for key, value := range mapl {
fmt.Println("key: ", key, " - value: ", value)
}
}
<file_sep>/exercism/beer-song/beer.go
package beer
import (
"errors"
"fmt"
"strings"
)
const (
upperBound int = 99
lowerBound int = 0
)
const (
v0 = `No more bottles of beer on the wall, no more bottles of beer.\n
Go to the store and buy some more, 99 bottles of beer on the wall.\n`
v1 = `1 bottle of beer on the wall, 1 bottle of beer.\n
Take it down and pass it around, no more bottles of beer on the wall.\n`
v2 = `%v bottles of beer on the wall, %v bottles of beer.\n
\Take one down and pass it around, 1 bottle of beer on the wall.\n`
vN = `%v bottles of beer on the wall, %v bottles of beer.\n
Take one down and pass it around, %v bottles of beer on the wall.\n`
)
// Verse returns the verse based on the input bottle.
func Verse(bottle int) (string, error) {
if bottle < lowerBound || bottle > upperBound {
return "", errors.New("invalid number of bottles")
}
if bottle == 0 {
return v0, nil
}
if bottle == 1 {
return v1, nil
}
if bottle == 2 {
return fmt.Sprintf(v2, bottle, bottle), nil
}
return fmt.Sprintf(vN, bottle, bottle, bottle-1), nil
}
// Verses returns a range from [upper] to [lower] of verses.
func Verses(upper, lower int) (string, error) {
if lower < lowerBound || upper > upperBound || lower > upper {
return "", errors.New("invalid bound(s)")
}
verses := make([]string, upper-lower+1)
for i := upper; i >= lower; i-- {
v, err := Verse(i)
if err != nil {
return "", err
}
verses = append(verses, v, "\n")
}
return strings.Join(verses, ""), nil
}
// Song returns the entire song.
func Song() string {
s, _ := Verses(99, 0)
return s
}
<file_sep>/tour/methods-interfaces/11-errors-Sqrt.go
package main
import (
"fmt"
"math"
)
type ErrNegativeSqrt float64
func (e ErrNegativeSqrt) Error() string {
return fmt.Sprintf("cannot Sqrt negative number: %f", float64(e))
}
const e = 1e-8
func Sqrt(x float64) (float64, error) {
if x < 0 {
return 0, ErrNegativeSqrt(x)
}
if x == 0 {
return 0, nil
}
z := float64(1)
for {
oldZ := z
z -= (z*z - x) / (2 * z)
if math.Abs(z-oldZ) < e {
return z, nil
}
}
return z, nil
}
func main() {
fmt.Println(Sqrt(2))
fmt.Println(math.Sqrt(2))
fmt.Println(Sqrt(-2))
}
<file_sep>/gophercises/03-choose-your-own-adventure/main.go
package main
import (
"errors"
"html/template"
"io/ioutil"
"log"
"net/http"
"strings"
"github.com/nguyenthanh1290/gophercises/03-choose-your-own-adventure/story"
)
var templates = template.Must(template.ParseFiles("index.html", "story.html"))
var stories map[string]story.Story
var storyNames []string
func main() {
var err error
stories, err = loadStories("./story/")
if err != nil {
log.Fatal(err)
}
storyNames, err = listStories(stories)
if err != nil {
log.Fatal(err)
}
http.HandleFunc("/", rootHandler)
http.HandleFunc("/story", storyHandler)
log.Fatal(http.ListenAndServe(":8080", nil))
}
func loadStories(dirname string) (map[string]story.Story, error) {
stories := make(map[string]story.Story)
files, err := ioutil.ReadDir(dirname)
if err != nil {
return nil, err
}
for _, f := range files {
if strings.HasSuffix(f.Name(), ".json") {
st, _ := loadStory(dirname + f.Name())
stories[f.Name()] = st
}
}
return stories, nil
}
func loadStory(name string) (story.Story, error) {
data, err := ioutil.ReadFile(name)
if err != nil {
return nil, err
}
st, err := story.New(data)
if err != nil {
return nil, err
}
return st, nil
}
func listStories(stories map[string]story.Story) ([]string, error) {
if len(stories) < 1 {
return nil, errors.New("Empty stories")
}
names := make([]string, len(stories))
i := 0
for k := range stories {
names[i] = strings.TrimRight(k, ".json")
i++
}
return names, nil
}
// rootHandler handles all requests to website root /
// where the user can choose a story to begin with
func rootHandler(w http.ResponseWriter, r *http.Request) {
templates.ExecuteTemplate(w, "index.html", storyNames)
}
// storyHandler handles requests matching /story?name=[story name]&arc=[next arc]
// Ex: /story?name=gopher&arc=new-york
func storyHandler(w http.ResponseWriter, r *http.Request) {
query := r.URL.Query()
name := query.Get("name")
if name == "" {
http.Redirect(w, r, "/", http.StatusNotFound)
}
name += ".json"
arc := query.Get("arc")
if arc == "" {
arc = story.BeginArc
}
templates.ExecuteTemplate(w, "story.html", stories[name][arc])
}
<file_sep>/tour/first-class-functions/first-class-functions.go
package main
import "fmt"
func main() {
fmt.Println("First class functions")
s1 := Student{
Firstname: "Steven",
Lastname: "Swachb",
Age: 33,
Grade: "12",
}
s2 := Student{
Firstname: "Steven",
Lastname: "Tran",
Age: 30,
Grade: "10",
}
s3 := Student{
Firstname: "Jonh",
Lastname: "Awd",
Age: 30,
Grade: "10",
}
s := []Student{s1, s2, s3}
f := filter(s, func(s Student) bool {
if s.Age == 30 {
return true
}
return false
})
fmt.Println("Filter")
fmt.Println(f)
a := []int{5, 6, 7, 8, 9}
r := iMap(a, func(n int) int {
return n * 5
})
fmt.Println("Mapping")
fmt.Println(r)
}
// Student stores a student data
type Student struct {
Firstname string
Lastname string
Age int
Grade string
}
func filter(s []Student, f func(s Student) bool) []Student {
var r []Student
for _, v := range s {
if f(v) {
r = append(r, v)
}
}
return r
}
func iMap(s []int, f func(int) int) []int {
var r []int
for _, v := range s {
r = append(r, f(v))
}
return r
}
<file_sep>/exercism/clock/clock.go
// Package clock implements a clock that handles times without dates.
package clock
import "fmt"
// Clock defines the clock type with hour and minute.
type Clock struct {
hour, minute int
}
const minOfDay = 24 * 60
// New returns a new clock.
func New(h, m int) Clock {
totalMin := (minOfDay + (h*60+m)%minOfDay) % minOfDay
h = totalMin / 60
m = totalMin % 60
return Clock{h, m}
}
// Add returns a new clock after adding minutes to it.
func (c Clock) Add(m int) Clock {
return New(c.hour, c.minute+m)
}
// Subtract returns a new clock after subtracting minutes to it.
func (c Clock) Subtract(m int) Clock {
return New(c.hour, c.minute-m)
}
// String returns a string that represents the clock in format of [hh]:[mm].
func (c Clock) String() string {
return fmt.Sprintf("%02d:%02d", c.hour, c.minute)
}
<file_sep>/tour/methods-interfaces/06-methods-and-pointer-indirection.go
package main
import "fmt"
type Vertex struct {
X, Y float64
}
func (v *Vertex) Scale(f float64) {
v.X = v.X * f
v.Y = v.Y * f
}
func ScaleFunc(v *Vertex, f float64) {
v.X = v.X * f
v.Y = v.Y * f
}
func main() {
v := Vertex{3, 4}
v.Scale(2)
// even though v is a value and not a pointer, the method with the pointer receiver is called automatically.
// That is, as a convenience, Go interprets the statement v.Scale(2) as (&v).Scale(2) since the Scale method has a pointer receiver.
// ScaleFunc(v, 10) // Compile error!
ScaleFunc(&v, 10)
// functions with a pointer argument must take a pointer
p := &Vertex{4, 3}
p.Scale(3)
ScaleFunc(p, 8)
fmt.Println(v, p)
}
<file_sep>/codility/03-time-complexity/perm-missing-elem/perm_missing_elem.go
package main
import (
"fmt"
)
func Solution(A []int) int {
if len(A) == 0 {
return 1
}
sumA := 0
for _, v := range A {
sumA += v
}
n := len(A) + 1
sumM := n * (n + 1) / 2
return sumM - sumA
}
func main() {
fmt.Println(Solution([]int{2, 3, 1, 5}))
}
// https://app.codility.com/programmers/lessons/3-time_complexity/perm_missing_elem/
// https://app.codility.com/demo/results/trainingJSDCQ6-8XK/
// Detected time complexity:
// O(N)<file_sep>/patterns/concurrency/06/time_out.go
package main
import (
"fmt"
"math/rand"
"time"
)
func boring(msg string) <-chan string {
c := make(chan string)
go func() {
for i := 0; ; i++ {
c <- fmt.Sprintf("%s %d", msg, i)
time.Sleep(time.Duration(rand.Intn(1e3)) * time.Millisecond)
}
}()
return c
}
func main() {
c := boring("Long")
for {
select {
case s := <-c:
fmt.Println(s)
case <-time.After(500 * time.Millisecond):// timeout for each message, that's why it takes too long to time out.
fmt.Println("You're too slow.")
return
}
}
}
// https://talks.golang.org/2012/concurrency.slide#35
<file_sep>/codility/others/bulbs/bulbs.go
package main
import (
"fmt"
"sort"
)
func main() {
a1 := []int{2, 1, 3, 5, 4}
a2 := []int{2, 3, 4, 1, 5}
a3 := []int{1, 3, 4, 2, 5}
a4 := []int{1, 2, 6, 3, 4, 5}
fmt.Println(Solution(a1))
fmt.Println(Solution(a2))
fmt.Println(Solution(a3))
fmt.Println(Solution(a4))
}
func Solution(A []int) int {
count := 0
for i := 0; i < len(A); i++ {
arr := A[:i+1]
if areAllBulbsTurnedOn(arr) {
count++
}
}
return count
}
// given the input condition: the elements of A are all distinct,
// we can verify if all the bulbs are turned of by sorting and
// then check if the first element is 1 and
// the last element equals to the total number of the array.
func areAllBulbsTurnedOn(arr []int) bool {
sort.Ints(arr)
if arr[0] == 1 && arr[len(arr)-1] == len(arr) {
return true
}
return false
}
<file_sep>/codility/09-max-slice-problem/max-slice-sum/max_slice_sum.go
package main
import (
"fmt"
)
// https://app.codility.com/demo/results/trainingPESXMD-AA7/
// Detected time complexity: O(N)
func max(A []int) int {
maxSlice := A[0]
maxEnding := A[0]
for i := 1; i < len(A); i++ {
if A[i] > maxEnding+A[i] {
maxEnding = A[i]
} else {
maxEnding += A[i]
}
if maxEnding > maxSlice {
maxSlice = maxEnding
}
}
return maxSlice
}
func main() {
fmt.Println(max([]int{3, 2, -6, 4, 0})) // 5
fmt.Println(max([]int{-10})) // -10
fmt.Println(max([]int{-10, -3})) // -3
fmt.Println(max([]int{5, 1})) // 6
fmt.Println(max([]int{-2, 1})) // 1
fmt.Println(max([]int{1, 3})) // 4
}
<file_sep>/tour/concurrency/temp.go
package main
import (
"sync"
"fmt"
)
type FreqMap map[rune]int
func ConcurrentFrequency(strs []string) FreqMap {
var counter = struct {
sync.Mutex
freq FreqMap
}{freq: map[rune]int{}}
var wg sync.WaitGroup
wg.Add(len(strs))
for _, s := range strs {
go func(s string) {
for _, r := range s {
counter.Lock()
counter.freq[r]++
counter.Unlock()
}
wg.Done()
}(s)
}
wg.Wait()
return counter.freq
}
func main() {
var f = ConcurrentFrequency([]string{euro, dutch, us})
fmt.Println(f)
}
var (
euro = `Fre<NAME>
Tochter aus Elysium,
Wir betreten feuertrunken,
Himmlische, dein Heiligtum!
Deine Zauber binden wieder
Was die Mode streng geteilt;
Alle Menschen werden Brüder,
Wo dein sanfter Flügel weilt.`
dutch = `<NAME>
ben ik, van Duitsen bloed,
den vaderland getrouwe
blijf ik tot in den dood.
Een Prinse van Oranje
ben ik, vrij, onverveerd,
den Koning van Hispanje
heb ik altijd geëerd.`
us = `O say can you see by the dawn's early light,
What so proudly we hailed at the twilight's last gleaming,
Whose broad stripes and bright stars through the perilous fight,
O'er the ramparts we watched, were so gallantly streaming?
And the rockets' red glare, the bombs bursting in air,
Gave proof through the night that our flag was still there;
O say does that star-spangled banner yet wave,
O'er the land of the free and the home of the brave?`
)<file_sep>/codility/07-stacks-queues/fish/fish.go
package fish
// https://app.codility.com/demo/results/trainingCESQ49-XGY/
// Detected time complexity:
// O(N)
func Solution(A []int, B []int) int {
survivals := 0
down := []int{}
for i, v := range A {
if B[i] == 0 {
if len(down) == 0 {
survivals++
} else {
j := len(down) - 1
for j >= 0 {
if down[j] > v {
break
}
j--
}
if j < 0 {
down = []int{}
survivals++
} else {
down = down[:j+1]
}
}
} else {
down = append(down, v)
}
}
survivals += len(down)
return survivals
}<file_sep>/tour/concurrency/20-Pi.go
// http://www.mathscareers.org.uk/article/calculating-pi/
// Calculating Pi (π) using infinite series
// Gregory-Leibniz Series
package main
import (
"fmt"
"math"
)
func main() {
fmt.Println(pi(5000))
}
func pi(n int) float64 {
c := make(chan float64)
for i := 0; i <= n; i++ {
go term(c, float64(i))
}
pi := 0.0
for i := 0; i <= n; i++ {
pi += <-c
}
return pi
}
func term(c chan float64, k float64) {
c <- 4 * math.Pow(-1, k) / (2*k + 1)
}
<file_sep>/codility/02-arrays/cyclic-rotation/cyclic_rotation.go
package main
import (
"fmt"
)
func Solution(A []int, K int) []int {
if K == 0 || len(A) == 0 || len(A) == 1 {
return A
}
if K > len(A) {
K = K % len(A)
}
arr := A[len(A)-K:]
arr = append(arr, A[:len(A)-K]...)
return arr
}
func main() {
fmt.Println(Solution([]int{4, 6, 0, 0, -2, 10}, 15))
}
// https://app.codility.com/programmers/lessons/2-arrays/cyclic_rotation/<file_sep>/exercism/acronym/acronym.go
// Package acronym provides a function that converts a pharse to its ancronym.
package acronym
import (
"regexp"
"strings"
)
// Abbreviate converts a pharse to its ancronym.
func Abbreviate(s string) string {
reg := regexp.MustCompile(`\b[a-zA-Z]`)
abbr := reg.FindAllString(s, -1)
return strings.ToUpper(strings.Join(abbr, ""))
}
<file_sep>/tour/basic/02-variables.go
package main
import "fmt"
// A var statement can be at package or function level.
var c, python, java bool
func main() {
fmt.Println(c, python, java)
// A var declaration can include initializers, one per variable.
// If an initializer is present, the type can be omitted; the variable will take the type of the initializer.
fmt.Println("integer:")
var a int = 1
var b, c int
b = 2
c = 3
fmt.Println(a)
fmt.Println(b + c)
fmt.Println("floating point number:")
var d float64 = 9.99
fmt.Println(d)
var str string = "This is a string"
fmt.Println(str)
// Shorthand Declaration
// Inside a function, the := short assignment statement can be used in place of a var declaration with implicit type.
// foo := "bar" is equivalent to var foo string = "bar"
e := 9
f := "golang"
g := 923.2
fmt.Println(e)
fmt.Println(f)
fmt.Println(g)
// Outside a function, every statement begins with a keyword (var, func, and so on)
// and so the := construct is not available.
}
<file_sep>/exercism/allergies/allergies.go
// Package allergies provides functions that help determining allergy.
package allergies
var allergenDb = map[string]uint{
"eggs": 1,
"peanuts": 2,
"shellfish": 4,
"strawberries": 8,
"tomatoes": 16,
"chocolate": 32,
"pollen": 64,
"cats": 128,
}
// Allergies returns a full list of allergies based on input score with the tesed database.
func Allergies(score uint) (allergens []string) {
for k, v := range allergenDb {
if score&v != 0 {
allergens = append(allergens, k)
}
}
return
}
// AllergicTo determines whether the person is allergic to the given allergen with the given score.
func AllergicTo(score uint, allergen string) bool {
if v, prs := allergenDb[allergen]; prs {
if score&v != 0 {
return true
}
}
return false
}
<file_sep>/codility/05-prefix-sums/min-avg/min_avg.go
package minavg
// Detected time complexity:
// O(N ** 2)
func Solution1(A []int) int {
result := 0
minAvg := 100000.0
for i := 0; i < len(A)-1; i++ {
sum := float64(A[i])
divisor := 2.0
for j := i + 1; j < len(A); j++ {
sum += float64(A[j])
avg := sum / divisor
if avg < minAvg {
minAvg = avg
result = i
}
divisor++
}
}
return result
}
// https://app.codility.com/demo/results/training75V4F8-ETS/
// Detected time complexity:
// O(N)
// The slice contains at least two elements =>
// we only need to check for slices of length 2 and 3,
// because slices of length 4 are actually 2 slices of length 2.
func Solution(A []int) int {
minAvg := float64(A[0]+A[1]) / 2.0
result := 0
for i := 0; i < len(A)-1; i++ {
avg := float64(A[i]+A[i+1]) / 2.0
if avg < minAvg {
minAvg = avg
result = i
}
}
for i := 0; i < len(A)-2; i++ {
avg := float64(A[i]+A[i+1]+A[i+2]) / 3.0
if avg < minAvg {
minAvg = avg
result = i
}
}
return result
}
<file_sep>/codility/07-stacks-queues/brackets/brackets.go
package brackets
// https://app.codility.com/c/run/trainingNCAFFJ-FF3/
// https://app.codility.com/demo/results/trainingNCAFFJ-FF3/
// Detected time complexity:
// O(N)
func Solution(S string) int {
if len(S) == 0 {
return 1
}
stack := []rune{}
for _, v := range S {
switch v {
case '(', '{', '[':
stack = append(stack, v)
case ')', '}', ']':
if len(stack) == 0 {
return 0
}
pair := string(stack[len(stack)-1]) + string(v)
if pair != "()" && pair != "{}" && pair != "[]" {
return 0
}
stack = stack[:len(stack)-1]
default:
return 0
}
}
if len(stack) > 0 {
return 0
}
return 1
}
<file_sep>/codility/05-prefix-sums/genomic-range-query/genomic_test.go
package genomic
import (
"testing"
)
func TestSolution(t *testing.T) {
for _, c := range testCases {
output := Solution(c.dna, c.p, c.q)
if len(output) != len(c.expected) {
t.Fatalf("FAIL:\nDNA: %v\nQuery: %v %v\nexpected: %v\ngot: %v", c.dna, c.p, c.q, c.expected, output)
}
for i, v := range output {
if v != c.expected[i] {
t.Fatalf("FAIL:\nDNA: %v\nQuery: %v %v\nexpected: %v\ngot: %v", c.dna, c.p, c.q, c.expected, output)
}
}
t.Logf("PASS: %v", c.dna)
}
}
func BenchmarkSolution(b *testing.B) {
for i := 0; i < b.N; i++ {
for _, c := range testCases {
Solution(c.dna, c.p, c.q)
}
}
}
var testCases = []struct {
dna string
p []int
q []int
expected []int
}{
{
dna: "CAGCCTA",
p: []int{2, 5, 0},
q: []int{4, 5, 6},
expected: []int{2, 4, 1},
},
{
dna: "C",
p: []int{0},
q: []int{0},
expected: []int{2},
},
{
dna: "CA",
p: []int{0},
q: []int{1},
expected: []int{1},
},
{
dna: "CC",
p: []int{0, 0, 1},
q: []int{1, 0, 1},
expected: []int{2, 2, 2},
},
{
dna: "AAAC",
p: []int{0, 0, 1, 3, 2},
q: []int{1, 2, 3, 3, 3},
expected: []int{1, 1, 1, 2, 1},
},
}
<file_sep>/exercism/README.md
https://exercism.io<file_sep>/patterns/options/options.go
package options
import (
"context"
"fmt"
"net/http"
"time"
)
const (
defaultTimeout = 1 * time.Second
)
func main() {
fmt.Println("Options pattern")
tr := &http.Transport{
MaxIdleConns: 10,
IdleConnTimeout: 30 * time.Second,
DisableCompression: true,
}
client := &http.Client{Transport: tr}
u := NewContext(
WithUsername("thanhn"),
WithPassword("<PASSWORD>"),
WithClient(client),
WithTimeout(defaultTimeout),
)
fmt.Printf("%#v", u)
}
type Context struct {
ctx context.Context
client *http.Client
username string
password string
timeout time.Duration
}
// NewContext creates a new Context with the provided options
func NewContext(opts ...ContextOption) *Context {
// Here we can do any initialization for all options, then the provided parameters can overwrite them.
uCtx := Context{}
for _, o := range opts {
o(&uCtx)
}
return &uCtx
}
type ContextOption func(*Context)
// WithTimeout sets the context timeout
func WithTimeout(ttl time.Duration) ContextOption {
return func(c *Context) {
c.timeout = ttl
var cancel func()
c.ctx, cancel = context.WithTimeout(context.Background(), ttl)
tick := time.NewTimer(ttl)
go func() {
select {
case <-c.ctx.Done():
cancel()
case <-tick.C:
cancel()
}
}()
}
}
// WithClient set an *http.Client to be used by the context
func WithClient(client *http.Client) ContextOption {
return func(c *Context) {
c.client = client
}
}
// WithUsername is an option that sets the username for remote server collector connections
func WithUsername(username string) ContextOption {
return func(c *Context) {
c.username = username
}
}
// WithPassword is an option that sets the password for remote server collector connections
func WithPassword(password string) ContextOption {
return func(c *Context) {
c.password = password
}
}
// FromContext sets a derived context
func FromContext(ctx context.Context) ContextOption {
return func(c *Context) {
c.ctx = context.WithValue(c.ctx, "context", ctx)
}
}
<file_sep>/gophercises/03-choose-your-own-adventure/README.md
Exercise details can be found here: https://gophercises.com/exercises/cyoa
Recommand to check this well guided [doc](https://golang.org/doc/articles/wiki/) before doing the exercise to have a good base knowledge of the net/http package.
## Skills developed
- net/http
- html/template
- encoding/json
- Decoding/Unmarshal
- strings
### Issue
<file_sep>/learn-go-with-tests/fundamentals/go.mod
module tdd
go 1.15
<file_sep>/gophercises/03-choose-your-own-adventure/story/story.go
package story
import (
"encoding/json"
)
type Story map[string]Chapter
type Chapter struct {
Title string `json:"title"`
Paragraphs []string `json:"story"`
Options []Option `json:"options"`
}
type Option struct {
Text string `json:"text"`
Arc string `json:"arc"`
}
const BeginArc = "intro"
func New(data []byte) (Story, error) {
var story Story
err := json.Unmarshal(data, &story)
if err != nil {
return nil, err
}
return story, nil
}
<file_sep>/codility/03-time-complexity/tape-quilibrium/tape_quilibrium.go
package main
import (
"fmt"
"math"
)
func Solution(A []int) int {
min := math.MaxInt32
total := sum(A...)
lSum := 0
for i := 0; i < len(A)-1; i++ {
lSum += A[i]
rSum := total - lSum
diff := abs(lSum - rSum)
if diff < min {
min = diff
}
}
return min
}
func abs(n int) int {
return int(math.Abs(float64(n)))
}
func sum(n ...int) int {
sum := 0
for _, v := range n {
sum += v
}
return sum
}
func main() {
fmt.Println(Solution([]int{3, 1, 2, 4, 3}))
}
// https://app.codility.com/programmers/lessons/3-time_complexity/tape_equilibrium/
// https://app.codility.com/demo/results/training5MXBJQ-SRA/
// Detected time complexity:
// O(N)<file_sep>/exercism/reverse-string/reverse_string.go
package reverse
import (
"strings"
)
// String reverses the input.
func String(s string) string {
var builder strings.Builder
runes := []rune(s)
for i := len(runes) - 1; i >= 0; i-- {
builder.WriteRune(runes[i])
}
return builder.String()
}
<file_sep>/patterns/concurrency/04/restoring_sequencing.go
package main
import (
"fmt"
"math/rand"
"time"
)
// Message defines a message type that contains a channel for the reply.
type Message struct {
str string
wait chan bool
}
// https://talks.golang.org/2012/concurrency.slide#29
// Send a channel on a channel, making goroutine wait its turn.
// Receive all messages, then enable them again by sending on a private channel.<file_sep>/codility/others/max-repeated-square-root/square_root.go
package main
import (
"fmt"
"math"
"sort"
)
func maxRepeated(A, B int) int {
lower := int(math.Ceil(math.Sqrt(float64(A))))
upper := int(math.Floor(math.Sqrt(float64(B))))
sqrts := []int{}
for i := lower; i <= upper; i++ {
sqrts = append(sqrts, primitiveSqrt(i))
}
sort.Ints(sqrts)
return sqrts[len(sqrts)-1]
}
func primitiveSqrt(n int) int {
steps := 0
p := int(math.Sqrt(float64(n)))
if p*p == n {
steps++
return steps + primitiveSqrt(p)
}
return steps
}
func main() {
fmt.Println(maxRepeated(10, 20))
fmt.Println(maxRepeated(6000, 7000))
}
<file_sep>/exercism/collatz-conjecture/collatz_conjecture.go
package collatzconjecture
import "errors"
// CollatzConjecture takes any [n] positive number and returns the number of steps required to reach 1 by repeating divide [n] by 2, if [n] is even, else multiply [n] by 3 and add 1.
func CollatzConjecture(n int) (int, error) {
if n <= 0 {
return 0, errors.New("positive integer is required")
}
steps := 0
for n != 1 {
if n%2 == 0 {
n = n / 2
} else {
n = n*3 + 1
}
steps++
}
return steps, nil
}
<file_sep>/exercism/etl/etl.go
package etl
import (
"strings"
)
// Transform returns an ETL of input.
func Transform(in map[int][]string) (out map[string]int) {
out = map[string]int{}
for k, v := range in {
for _, e := range v {
out[strings.ToLower(e)] = k
}
}
return
}
<file_sep>/gophercises/02-url-shortener/README.md
Exercise details can be found here: https://gophercises.com/exercises/urlshort
However, I recommend to check this Go doc first, https://golang.org/doc/articles/wiki/. By following the doc to build a Web App, you will have enough knowledge about http package to complete this exercise.
## Skills developed
* net/http package
* yaml
### Issue
"panic: yaml: line 2: found character that cannot start any token"
Fix:
- make sure there is no tab character in your yaml text
- that includes, do not indent the lines when declaring the variable storing the yaml text
<file_sep>/exercism/grade-school/grade_school.go
package school
import (
"sort"
)
// Grade is a struct with a level and a slice of students.
type Grade struct {
level int
students []string
}
// School is a map with grade as key and slice of students as value.
type School map[int][]string
// New returns an empty school.
func New() *School {
return &School{}
}
// Add adds a student to appropriate grade.
func (s *School) Add(name string, level int) {
if stus, prs := (*s)[level]; prs {
stus = append(stus, name)
(*s)[level] = stus
} else {
(*s)[level] = []string{name}
}
}
// Grade returns all students enrolled.
func (s *School) Grade(level int) []string {
if _, prs := (*s)[level]; prs {
return (*s)[level]
}
return []string{}
}
// Enrollment returns a sorted list of all students in all grades.
func (s *School) Enrollment() []Grade {
ret := []Grade{}
levels := []int{}
for k := range *s {
levels = append(levels, k)
}
sort.Ints(levels)
for _, l := range levels {
stus := (*s)[l]
sort.Strings(stus)
ret = append(ret, Grade{l, stus})
}
return ret
}
<file_sep>/crypto/crypto.go
package crypto
import (
"bytes"
"crypto/aes"
"crypto/cipher"
"crypto/rand"
"errors"
"io"
)
// Encryption/decryption errors.
var (
ErrPlaintextEmpty = errors.New("plaintext is empty")
ErrCiphertextTooShort = errors.New("ciphertext is too short")
ErrCiphertextNotMultipleOfBlockSize = errors.New("ciphertext is not a multiple of the block size")
ErrPlaintextNotMultipleOfBlockSize = errors.New("plaintext is not a multiple of the block size")
// ErrInvalidBlockSize indicates hash blocksize <= 0.
ErrInvalidBlockSize = errors.New("invalid blocksize")
// ErrInvalidPKCS7Data indicates bad input to PKCS7 pad or unpad.
ErrInvalidPKCS7Data = errors.New("invalid PKCS7 data (empty or not padded)")
// ErrInvalidPKCS7Padding indicates PKCS7 unpad fails to bad input.
ErrInvalidPKCS7Padding = errors.New("invalid padding on input")
)
// Encrypt encrypts the given byte slice using AES-CBC algorithm.
func Encrypt(plaintext []byte, key []byte) ([]byte, error) {
if len(plaintext) == 0 {
return nil, ErrPlaintextEmpty
}
plaintext, err := pkcs7Pad(plaintext, aes.BlockSize)
if err != nil {
return nil, err
}
if len(plaintext)%aes.BlockSize != 0 {
return nil, ErrPlaintextNotMultipleOfBlockSize
}
block, err := aes.NewCipher(key)
if err != nil {
return nil, err
}
// The IV needs to be unique, but not secure. Therefore it's common to
// include it at the beginning of the ciphertext.
ciphertext := make([]byte, aes.BlockSize+len(plaintext))
iv := ciphertext[:aes.BlockSize]
if _, err := io.ReadFull(rand.Reader, iv); err != nil {
return nil, err
}
mode := cipher.NewCBCEncrypter(block, iv)
mode.CryptBlocks(ciphertext[aes.BlockSize:], plaintext)
return ciphertext, nil
}
// Decrypt decrypts the AES-CBC algorithm encrypted cipher text.
func Decrypt(ciphertext []byte, key []byte) ([]byte, error) {
if len(ciphertext) < aes.BlockSize {
return nil, ErrCiphertextTooShort
}
block, err := aes.NewCipher(key)
if err != nil {
return nil, err
}
// Remove the embedded IV.
iv := ciphertext[:aes.BlockSize]
ciphertext = ciphertext[aes.BlockSize:]
// CBC mode always works in whole blocks.
if len(ciphertext)%aes.BlockSize != 0 {
return nil, ErrCiphertextNotMultipleOfBlockSize
}
mode := cipher.NewCBCDecrypter(block, iv)
plaintext := make([]byte, len(ciphertext))
mode.CryptBlocks(plaintext, ciphertext)
plaintext, err = pkcs7Unpad(plaintext, aes.BlockSize)
if err != nil {
return nil, err
}
return plaintext, nil
}
// pkcs7Pad right-pads the given byte slice with 1 to n bytes, where
// n is the block size. The size of the result is x times n, where x
// is at least 1.
func pkcs7Pad(b []byte, blocksize int) ([]byte, error) {
if blocksize <= 0 {
return nil, ErrInvalidBlockSize
}
if len(b) == 0 {
return nil, ErrInvalidPKCS7Data
}
n := blocksize - (len(b) % blocksize)
pb := make([]byte, len(b)+n)
copy(pb, b)
copy(pb[len(b):], bytes.Repeat([]byte{byte(n)}, n))
return pb, nil
}
// pkcs7Unpad validates and unpads data from the given bytes slice.
// The returned value will be 1 to n bytes smaller depending on the
// amount of padding, where n is the block size.
func pkcs7Unpad(b []byte, blocksize int) ([]byte, error) {
if blocksize <= 0 {
return nil, ErrInvalidBlockSize
}
if len(b) == 0 {
return nil, ErrInvalidPKCS7Data
}
if len(b)%blocksize != 0 {
return nil, ErrInvalidPKCS7Padding
}
c := b[len(b)-1]
n := int(c)
if n == 0 || n > len(b) {
return nil, ErrInvalidPKCS7Padding
}
for i := 0; i < n; i++ {
if b[len(b)-n+i] != c {
return nil, ErrInvalidPKCS7Padding
}
}
return b[:len(b)-n], nil
}
<file_sep>/tour/doc-play/README.md
https://golang.org/doc/play/<file_sep>/exercism/triangle/triangle.go
// Package triangle provides a function that determines triangle type.
package triangle
import "math"
// Kind defines the triangle type.
type Kind int
// Different kinds of triangle
// - NaT: not a triangle
// - Equ: equilateral
// - Iso: isosceles
// - Sca: scalene
const (
NaT Kind = 1 << iota
Equ
Iso
Sca
)
// KindFromSides takes the given sides and returns the type of a triangle they can form.
func KindFromSides(a, b, c float64) Kind {
// for _, v := range []float64{a, b, c} {
// if v <= 0 || math.IsNaN(v) || math.IsInf(v, 1) {
// return NaT
// }
// }
if a <= 0 || b <= 0 || c <= 0 || math.IsNaN(a+b+c) || math.IsInf(a+b+c, 1) {
return NaT
}
if (a+b < c) || (a+c < b) || (b+c < a) {
return NaT
}
if a == b && b == c {
return Equ
}
if a == b || a == c || b == c {
return Iso
}
return Sca
}
<file_sep>/exercism/accumulate/accumulate.go
package accumulate
// Accumulate returns a new collection containing the result of applying that operation to each element of the input collection.
func Accumulate(in []string, fn func(string) string) []string {
out := make([]string, len(in))
for i, e := range in {
out[i] = fn(e)
}
return out
}
<file_sep>/gophercises/03-choose-your-own-adventure/story/story_test.go
package story
import (
"testing"
)
func TestNew(t *testing.T) {
t.Error("Failed")
}<file_sep>/tour/basic/05-switch.go
package main
import (
"fmt"
"runtime"
"time"
)
func main() {
fmt.Print("Go runs on ")
switch os := runtime.GOOS; os {
case "darwin":
fmt.Println("OS X.")
case "linux":
fmt.Println("Linux.")
default:
// freebsd, openbsd,
// plan9, windows...
fmt.Printf("%s.", os)
}
fmt.Println("\n")
// switch evaluation order
today := time.Now().Weekday()
fmt.Println("Today is: ", today)
fmt.Println("When's Saturday?")
switch time.Saturday {
case today + 0:
fmt.Println("Today.")
case today + 1:
fmt.Println("Tomorrow.")
case today + 2:
fmt.Println("In two days.")
default:
fmt.Println("Too far away.")
}
fmt.Println("\n")
// Switch with no condition
t := time.Now()
fmt.Println("Now is: ", t)
switch {
case t.Hour() < 12:
fmt.Println("Good morning!")
case t.Hour() < 17:
fmt.Println("Good afternoon.")
default:
fmt.Println("Good evening.")
}
}
// Go only runs the selected case, not all the cases that follow.
// In effect, the break statement that is needed at the end of each case in those languages is provided automatically in Go.
// Another important difference is that Go's switch cases need not be constants,
// and the values involved need not be integers.
<file_sep>/crypto/ecdh/ecdh-curve25519.go
package ecdh
import (
"crypto"
"io"
"golang.org/x/crypto/curve25519"
)
type ecdhCurve25519 struct {
}
// GenerateKeyPair generates a pair of private and public key using entropy from rand.
func (e ecdhCurve25519) GenerateKeyPair(rand io.Reader) (crypto.PrivateKey, crypto.PublicKey, error) {
var private, public [32]byte
// Compute secret key.
_, err := rand.Read(private[:])
if err != nil {
return nil, nil, err
}
private[0] &= 248
private[31] &= 127
private[31] |= 64
// Compute public key.
curve25519.ScalarBaseMult(&public, &private)
return &private, &public, nil
}
// ComputeSharedSecret computes shared secret key.
func (e ecdhCurve25519) ComputeSharedSecret(private crypto.PrivateKey, peerPublic crypto.PublicKey) []byte {
var sharedSecret [32]byte
curve25519.ScalarMult(&sharedSecret, private.(*[32]byte), peerPublic.(*[32]byte))
return sharedSecret[:]
}
<file_sep>/tour/image-api/chessboard.go
package main
import (
"fmt"
"image"
"image/color"
"image/draw"
"image/png"
"os"
)
func main() {
new_png_file := "chessboard.png"
board_num_pixels := 240
myimage := image.NewRGBA(image.Rect(0, 0, board_num_pixels, board_num_pixels))
colors := make(map[int]color.RGBA, 2)
colors[0] = color.RGBA{0, 100, 0, 255} // green
colors[1] = color.RGBA{50, 205, 50, 255} // limegreen
index_color := 0
size_board := 8
size_block := int(board_num_pixels / size_board)
loc_x := 0
for curr_x := 0; curr_x < size_board; curr_x++ {
loc_y := 0
for curr_y := 0; curr_y < size_board; curr_y++ {
draw.Draw(myimage, image.Rect(loc_x, loc_y, loc_x+size_block, loc_y+size_block),
&image.Uniform{colors[index_color]}, image.ZP, draw.Src)
loc_y += size_block
index_color = 1 - index_color // toggle from 0 to 1 to 0 to 1 to ...
}
loc_x += size_block
index_color = 1 - index_color // toggle from 0 to 1 to 0 to 1 to ...
}
myfile, err := os.Create(new_png_file)
if err != nil {
panic(err.Error())
}
defer myfile.Close()
png.Encode(myfile, myimage) // ... save image
fmt.Println("firefox ", new_png_file) // view image issue : firefox /tmp/chessboard.png
}
<file_sep>/codility/12-euclidean-algorithm/chocolates/chocolates.go
package main
import (
"fmt"
)
// https://app.codility.com/demo/results/trainingE4989R-ZHY/
// Detected time complexity: O(log(N + M))
// Least common multiple
func eat(N, M int) int {
if 1 == M {
return N
}
if N == M {
return 1
}
a := N
b := M
for b != 0 {
a, b = b, a%b
}
return N / a
}
func main() {
fmt.Println(eat(10, 4)) // 5
fmt.Println(eat(10, 8)) // 5
fmt.Println(eat(100, 4)) // 25
fmt.Println(eat(100, 8)) // 25
fmt.Println(eat(1000, 4)) // 125
fmt.Println(eat(1000, 8)) // 125
fmt.Println(eat(10, 3)) // 10
fmt.Println(eat(10, 9)) // 10
fmt.Println(eat(7, 9)) // 7
fmt.Println(eat(2, 3)) // 2
fmt.Println(eat(2, 19)) // 2
fmt.Println(eat(2, 111119)) // 2
fmt.Println(eat(2, 1)) // 2
fmt.Println(eat(3, 1)) // 3
fmt.Println(eat(3, 2)) // 3
fmt.Println(eat(1000000000, 1)) // 1000000000
}
<file_sep>/exercism/raindrops/raindrops.go
// Package raindrops provides a function that convert raindrops to its appropriate sound.
package raindrops
import "fmt"
// Convert returns a sound based on the number of raindrops.
func Convert(drops int) string {
s := ""
if drops%3 == 0 {
s += "Pling"
}
if drops%5 == 0 {
s += "Plang"
}
if drops%7 == 0 {
s += "Plong"
}
if s == "" {
s = fmt.Sprintf("%v", drops)
}
return s
}
<file_sep>/codility/08-leader/dominator/dominator.go
package main
import (
"fmt"
)
// https://app.codility.com/demo/results/trainingK4BM6J-KRW/
// O(N)
func Solution(A []int) int {
if 0 == len(A) {
return -1
}
value := A[0]
size := 0
for _, a := range A {
if 0 == size {
size++
value = a
} else {
if a != value {
size--
} else {
size++
}
}
}
candidate := -1
if 0 != size {
candidate = value
}
index := -1
count := 0
for i, a := range A {
if a == candidate {
count++
if count > len(A)/2 {
return i
}
}
}
return index
}
func main() {
fmt.Println(Solution([]int{6, 8, 4, 6, 8, 6, 6})) // 6
fmt.Println(Solution([]int{1, 2, 19, 1, 4, 2, 1})) // -1
fmt.Println(Solution([]int{3, 4, 3, 2, 3, -1, 3, 3})) // 7
}
<file_sep>/exercism/isogram/isogram.go
// Package isogram provides functions that determines if a word or phrase is an isogram.
package isogram
import "unicode"
// IsIsogram returns true if input is an isogram, else returns false.
func IsIsogram(input string) bool {
dict := map[rune]int{}
for _, c := range input {
if c == ' ' || c == '-' {
continue
}
if _, prs := dict[unicode.ToUpper(c)]; prs {
return false
}
dict[unicode.ToUpper(c)]++
}
return true
}
<file_sep>/exercism/secret-handshake/secret_handshake.go
// Package secret provides functions that decodes the code to the appropriate sequence of events for a secret handshake.
package secret
const (
wink = 1 << iota
doubleBlink
closeEyes
jump
reverse
)
func reverseArray(input []string) {
for i, j := 0, len(input)-1; i < j; i, j = i+1, j-1 {
input[i], input[j] = input[j], input[i]
}
}
// Handshake returns a decoded handshake.
func Handshake(code uint) (output []string) {
if code&wink != 0 {
output = append(output, "wink")
}
if code&doubleBlink != 0 {
output = append(output, "double blink")
}
if code&closeEyes != 0 {
output = append(output, "close your eyes")
}
if code&jump != 0 {
output = append(output, "jump")
}
if code&reverse != 0 {
reverseArray(output)
}
return
}
<file_sep>/tour/basic/05-my-sqrt.go
package main
import (
"fmt"
"math"
)
func Sqrt(x float64) float64 {
z := float64(1)
for {
oldZ := z
z -= (z*z - x) / (2 * z)
fmt.Println("z = ", z)
if oldZ == z {
return z
}
}
return z
}
func main() {
x := float64(2222)
fmt.Println("My sqrt:", Sqrt(x))
fmt.Println("Go sqrt:", math.Sqrt(x))
}
<file_sep>/exercism/perfect-numbers/perfect_numbers.go
package perfect
import (
"errors"
)
// Classification represents the classification values for natural numbers.
type Classification int
// Classification values
const (
ClassificationDeficient Classification = iota
ClassificationPerfect
ClassificationAbundant
)
// ErrOnlyPositive is a customer error type.
var ErrOnlyPositive = errors.New("positive integer is required")
// Classify determines if a number is perfect, abundant, or deficient based on
// Nicomachus' (60 - 120 CE) classification scheme for natural numbers.
func Classify(n int64) (Classification, error) {
if n <= 0 {
return -1, ErrOnlyPositive
}
aSum := aliquotSum(n)
if aSum == n {
return ClassificationPerfect, nil
}
if aSum > n {
return ClassificationAbundant, nil
}
return ClassificationDeficient, nil
}
// The aliquot sum is the sum of the factors of a number not including the number itself.
func aliquotSum(n int64) (aSum int64) {
for i := int64(1); i < n; i++ {
if n%i == 0 {
aSum += i
}
}
return
}
<file_sep>/exercism/proverb/proverb.go
// Package proverb provides function to generate the "For Want of a Nail" proverb.
package proverb
import "fmt"
const (
line = "For want of a %s the %s was lost."
last = "And all for the want of a %s."
)
// Proverb generates the "For Want of a Nail" proverb base on input rhyme.
func Proverb(rhyme []string) []string {
if len(rhyme) == 0 {
return []string{}
}
proverb := make([]string, len(rhyme))
for i := 0; i < (len(rhyme) - 1); i++ {
proverb[i] = fmt.Sprintf(line, rhyme[i], rhyme[i+1])
}
proverb[len(rhyme)-1] = fmt.Sprintf(last, rhyme[0])
return proverb
}
<file_sep>/tour/temp.go
package main
import (
"fmt"
"math"
"strconv"
)
func main() {
fmt.Println(isPalindrome(-101))
fmt.Println(smallest(2))
fmt.Println(largest(2))
}
func smallest(n int) (minProduct int, factor1 int, factor2 int) {
lowerBound := int(math.Pow10(n - 1))
upperBound := int(math.Pow10(n) - 1)
minProduct = math.MaxInt32
for f1 := lowerBound; f1 <= upperBound; f1++ {
for f2 := lowerBound; f2 <= f1; f2++ {
p := f1 * f2
if p > minProduct {
break
}
if p < minProduct && isPalindrome(p) {
minProduct = p
factor1 = f1
factor2 = f2
}
}
}
return
}
func largest(n int) (maxProduct int, factor1 int, factor2 int) {
lowerBound := int(math.Pow10(n - 1))
upperBound := int(math.Pow10(n) - 1)
for f1 := upperBound; f1 >= lowerBound; f1-- {
for f2 := upperBound; f2 >= f1; f2-- {
p := f1 * f2
if p < maxProduct {
break
}
if p > maxProduct && isPalindrome(p) {
maxProduct = p
factor1 = f1
factor2 = f2
}
}
}
return
}
func isPalindrome(n int) bool {
return isPalindromeVc(n)
}
func isPalindromeVc(n int) bool {
reverse := 0
copyOfN := n
for copyOfN != 0 {
reverse = reverse*10 + copyOfN%10
copyOfN /= 10
}
return reverse == n
}
func isPalindromeVa(n int) bool {
if n < 0 {
n = -n
}
divisor := 1
for n/divisor >= 10 {
divisor *= 10
}
for n != 0 {
leading := n / divisor
trailing := n % 10
if leading != trailing {
return false
}
// Removing the leading and trailing digit from number.
n = (n % divisor) / 10
// Reducing divisor by a factor of 2 as 2 digits are dropped.
divisor /= 100
}
return true
}
func isPalindromeVb(n int) bool {
if n < 0 {
n = -n
}
s := strconv.Itoa(n)
l := len(s)
for i := 0; i < l/2; i++ {
if s[i] != s[l-1-i] {
return false
}
}
return true
}
<file_sep>/tour/basic/06-defer.go
package main
import "fmt"
func main() {
i := 0
i++
defer fmt.Println("defer i = ", i)
i++
fmt.Println("i = ", i)
}
// i= 2
// defer i = 1
// A defer statement defers the execution of a function until the surrounding function returns.
// The deferred call's arguments are evaluated immediately,
// but the function call is not executed until the surrounding function returns.
// 1. A deferred function's arguments are evaluated when the defer statement is evaluated.<file_sep>/codility/06-sorting/distinct/distinct.go
package distinct
import "sort"
// https://app.codility.com/demo/results/trainingJ2JGAF-HCV/
// Detected time complexity:
// O(N*log(N)) or O(N)
func Solution(A []int) int {
if len(A) == 0 {
return 0
}
sort.Ints(A)
result := 1
for i := 1; i < len(A); i++ {
if A[i] != A[i-1] {
result++
}
}
return result
}
// https://app.codility.com/demo/results/trainingTEP555-WWU/
// Detected time complexity:
// O(N)
func Solution1(A []int) int {
m := make(map[int]struct{})
for _, v := range A {
m[v] = struct{}{}
}
return len(m)
}
// by counting elements
// Notice that we have to know the range of the sorted values.
// If all the elements are in the set {0, 1, . . . , k},
// then the array used for counting should be of size k + 1
// The time complexity here is O(n + k).
// We need additional memory O(k) to count all the elements
func SolutionXXX(A []int, K int) int {
counter := make([]int, K + 1) //init all elements of the slice to 0 value
for _, v := range A {
counter[v]++
}
result := 0
for _, v := range counter {
if v > 0 {
result++
}
}
return result
}<file_sep>/patterns/concurrency/README.md
# go-concurrency-patterns
<file_sep>/exercism/prime-factors/prime_factors.go
package prime
// Factors returns the prime factors of the given natural number.
func Factors(n int64) []int64 {
r := make([]int64, 0)
for i := int64(2); i <= n; i++ {
for n%i == 0 {
r = append(r, i)
n /= i
}
}
return r
}
<file_sep>/tour/basic/10-array.go
package main
import "fmt"
func main() {
// int array
var arr [3]int
arr[0] = 1
arr[0] = 2
arr[0] = 3
// alternative syntax
arr2 := [4]int{3, 2, 5, 1}
fmt.Println(arr2)
//string array
var strArray [3]string
strArray[0] = "hello"
strArray[1] = "hi"
strArray[2] = "hey"
fmt.Println(strArray[2])
arrp := []int{1, 3, 6, 2, 6}
arrp = append(arrp, 23)
fmt.Println(arrp)
} | 1ca6b890b7ef8661725a39a9a32c9b4be8c43025 | [
"Markdown",
"Go Module",
"Go"
] | 121 | Go | wtrb/go | 66af8cbc2eb503ee0f049d0242165574874a6e27 | f2b4de8258b0b1680f0193b9949f0f14b5b18046 | |
refs/heads/main | <repo_name>putuprema/beeflix-aspnet<file_sep>/BeeFlix/Models/MovieDetailViewModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace BeeFlix.Models
{
public class MovieDetailViewModel
{
public Movie Movie { get; set; }
public PaginatedList<Episode> Episodes { get; set; }
}
}
<file_sep>/BeeFlix/Data/BeeFlixContext.cs
using BeeFlix.Models;
using Microsoft.EntityFrameworkCore;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace BeeFlix.Data
{
public class BeeFlixContext : DbContext
{
public BeeFlixContext(DbContextOptions<BeeFlixContext> options) : base(options)
{
}
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Movie>()
.HasOne(m => m.Genre)
.WithMany(g => g.Movies)
.OnDelete(DeleteBehavior.Cascade);
modelBuilder.Entity<Episode>()
.HasOne(e => e.Movie)
.WithMany(m => m.Episodes)
.OnDelete(DeleteBehavior.Cascade);
}
public DbSet<Genre> Genre { get; set; }
public DbSet<Movie> Movie { get; set; }
public DbSet<Episode> Episode { get; set; }
}
}
<file_sep>/BeeFlix/Data/DataSeeder.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.DependencyInjection;
using BeeFlix.Models;
namespace BeeFlix.Data
{
public static class DataSeeder
{
public static void Seed(IServiceProvider serviceProvider)
{
using (var context = new BeeFlixContext(
serviceProvider.GetRequiredService<DbContextOptions<BeeFlixContext>>()))
{
if (context.Genre.Any()) { return; }
context.Genre.AddRange(
new Genre
{
Name = "Drama",
Movies = new List<Movie>()
{
new Movie
{
Title = "The Shinning",
Description = "A family heads to an isolated hotel for the winter where a sinister presence influences the father into violence, while his psychic son sees horrific forebodings from both past and future.",
Rating = 4,
Photo = "/Storage/MoviePosters/the-shining.jpg"
},
new Movie
{
Title = "Parasite",
Description = "Greed and class discrimination threaten the newly formed symbiotic relationship between the wealthy Park family and the destitute Kim clan.",
Rating = 4,
Photo = "/Storage/MoviePosters/parasite.jpg"
},
new Movie
{
Title = "Mulan",
Description = "A young Chinese maiden disguises herself as a male warrior in order to save her father.",
Rating = 4,
Photo = "/Storage/MoviePosters/mulan.jpg"
},
new Movie
{
Title = "Joker",
Description = "In Gotham City, mentally troubled comedian <NAME> is disregarded and mistreated by society. He then embarks on a downward spiral of revolution and bloody crime. This path brings him face-to-face with his alter-ego: the Joker.",
Rating = 4,
Photo = "/Storage/MoviePosters/joker.jpg"
},
new Movie
{
Title = "Interstellar",
Description = "A team of explorers travel through a wormhole in space in an attempt to ensure humanity's survival.",
Rating = 4,
Photo = "/Storage/MoviePosters/interstellar.jpg"
},
new Movie
{
Title = "Whiplash",
Description = "A promising young drummer enrolls at a cut-throat music conservatory where his dreams of greatness are mentored by an instructor who will stop at nothing to realize a student's potential.",
Rating = 4,
Photo = "/Storage/MoviePosters/whiplash.jpg"
},
}
},
new Genre
{
Name = "Kids",
Movies = new List<Movie>()
{
new Movie
{
Title = "Hotel Transylvania",
Description = "Dracula, who operates a high-end resort away from the human world, goes into overprotective mode when a boy discovers the resort and falls for the count's teenaged daughter.",
Rating = 4,
Photo = "/Storage/MoviePosters/hotel-transylvania.jpg"
},
new Movie
{
Title = "Home Alone",
Description = "An eight-year-old troublemaker must protect his house from a pair of burglars when he is accidentally left home alone by his family during Christmas vacation.",
Rating = 4,
Photo = "/Storage/MoviePosters/home-alone.jpg"
},
new Movie
{
Title = "Monsters, Inc.",
Description = "In order to power the city, monsters have to scare children so that they scream. However, the children are toxic to the monsters, and after a child gets through, 2 monsters realize things may not be what they think.",
Rating = 4,
Photo = "/Storage/MoviePosters/monster-inc.jpg"
},
new Movie
{
Title = "Despicable Me",
Description = "When a criminal mastermind uses a trio of orphan girls as pawns for a grand scheme, he finds their love is profoundly changing him for the better.",
Rating = 4,
Photo = "/Storage/MoviePosters/despicable-me.jpg"
},
new Movie
{
Title = "Toy Story",
Description = "A cowboy doll is profoundly threatened and jealous when a new spaceman figure supplants him as top toy in a boy's room.",
Rating = 4,
Photo = "/Storage/MoviePosters/toy-story.jpg"
},
new Movie
{
Title = "Ratatouille",
Description = "A rat who can cook makes an unusual alliance with a young kitchen worker at a famous restaurant.",
Rating = 4,
Photo = "/Storage/MoviePosters/ratatouille.jpg"
},
}
},
new Genre
{
Name = "TV Shows",
Movies = new List<Movie>()
{
new Movie
{
Title = "Game of Thrones",
Description = "Nine noble families fight for control over the lands of Westeros, while an ancient enemy returns after being dormant for millennia.",
Rating = 4,
Photo = "/Storage/MoviePosters/game-of-thrones.jpg",
Episodes = new List<Episode>()
{
new Episode
{
Order = 1,
Title = "Winterfell"
},
new Episode
{
Order = 2,
Title = "A Knight of the Seven Kingdoms"
},
new Episode
{
Order = 3,
Title = "The Long Night"
},
new Episode
{
Order = 4,
Title = "The Last of the Starks"
},
new Episode
{
Order = 5,
Title = "The Bells"
},
new Episode
{
Order = 6,
Title = "The Iron Throne"
},
}
},
new Movie
{
Title = "<NAME>",
Description = "An animated series that follows the exploits of a super scientist and his not-so-bright grandson.",
Rating = 4,
Photo = "/Storage/MoviePosters/rick-and-morty.jpg",
Episodes = new List<Episode>()
{
new Episode
{
Order = 1,
Title = "Edge of Tomorty: Rick Die Rickpeat"
},
new Episode
{
Order = 2,
Title = "The Old Man and the Seat"
},
new Episode
{
Order = 3,
Title = "One Crew Over the Crewcoo's Morty"
},
new Episode
{
Order = 4,
Title = "Claw and Hoarder: Special Ricktim's Morty"
},
new Episode
{
Order = 5,
Title = "Rattlestar Ricklactica"
},
new Episode
{
Order = 6,
Title = "Never Ricking Morty"
},
new Episode
{
Order = 7,
Title = "Promortyus"
},
new Episode
{
Order = 8,
Title = "The Vat of Acid Episode"
},
new Episode
{
Order = 9,
Title = "Childrick of Mort"
},
new Episode
{
Order = 10,
Title = "Star Mort: Rickturn of the Jerri"
},
}
},
new Movie
{
Title = "The Office",
Description = "The story of an office that faces closure when the company decides to downsize its branches. A documentary film crew follow staff and the manager <NAME> as they continue their daily lives.",
Rating = 4,
Photo = "/Storage/MoviePosters/the-office.jpg",
Episodes = new List<Episode>()
{
new Episode
{
Order = 1,
Title = "New Guys"
},
new Episode
{
Order = 2,
Title = "Roy's Wedding"
},
new Episode
{
Order = 3,
Title = "Andy's Ancestry"
},
new Episode
{
Order = 4,
Title = "Work Bus"
},
new Episode
{
Order = 5,
Title = "Here Comes Treble"
},
new Episode
{
Order = 6,
Title = "The Boat"
},
}
},
new Movie
{
Title = "Stranger Things",
Description = "When a young boy disappears, his mother, a police chief and his friends must confront terrifying supernatural forces in order to get him back.",
Rating = 4,
Photo = "/Storage/MoviePosters/stranger-things.jpg",
Episodes = new List<Episode>()
{
new Episode
{
Order = 1,
Title = "Chapter One: Suzie, Do You Copy?"
},
new Episode
{
Order = 2,
Title = "Chapter Two: The Mall Rats"
},
new Episode
{
Order = 3,
Title = "Chapter Three: The Case of the Missing Lifeguard"
},
new Episode
{
Order = 4,
Title = "Chapter Four: The Sauna Test"
},
new Episode
{
Order = 5,
Title = "Chapter Five: The Flayed"
},
new Episode
{
Order = 6,
Title = "Chapter Six: E Pluribus Unum"
},
}
},
new Movie
{
Title = "The Simpsons",
Description = "The satiric adventures of a working-class family in the misfit city of Springfield.",
Rating = 4,
Photo = "/Storage/MoviePosters/the-simpsons.jpg",
Episodes = new List<Episode>()
{
new Episode
{
Order = 1,
Title = "Bart's Not Dead"
},
new Episode
{
Order = 2,
Title = "Heartbreak Hotel"
},
new Episode
{
Order = 3,
Title = "My Way or the Highway to Heaven"
},
new Episode
{
Order = 4,
Title = "Treehouse of Horror XXIX"
},
new Episode
{
Order = 5,
Title = "Baby You Can't Drive My Car"
},
new Episode
{
Order = 6,
Title = "From Russia Without Love"
},
}
},
new Movie
{
Title = "House of Cards",
Description = "A Congressman works with his equally conniving wife to exact revenge on the people who betrayed him.",
Rating = 4,
Photo = "/Storage/MoviePosters/house-of-cards.jpg",
Episodes = new List<Episode>()
{
new Episode
{
Order = 1,
Title = "Chapter 1"
},
new Episode
{
Order = 2,
Title = "Chapter 2"
},
new Episode
{
Order = 3,
Title = "Chapter 3"
},
new Episode
{
Order = 4,
Title = "Chapter 4"
},
new Episode
{
Order = 5,
Title = "Chapter 5"
},
new Episode
{
Order = 6,
Title = "Chapter 6"
},
}
},
}
}
);
context.SaveChanges();
}
}
}
}
<file_sep>/BeeFlix/Controllers/MovieController.cs
using BeeFlix.Data;
using BeeFlix.Models;
using Microsoft.AspNetCore.Mvc;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.Logging;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace BeeFlix.Controllers
{
public class MovieController : Controller
{
private readonly BeeFlixContext _context;
public MovieController(BeeFlixContext context)
{
_context = context;
}
public async Task<IActionResult> Index()
{
var genres = from g in _context.Genre select g;
genres = genres.Include(g => g.Movies);
return View(await genres.ToListAsync());
}
public async Task<IActionResult> Details(int id, int episodePage = 1)
{
var movieQuery = from m in _context.Movie select m;
movieQuery = movieQuery.Include(m => m.Genre);
var movie = await movieQuery.FirstOrDefaultAsync(m => m.Id == id);
if (movie == null)
{
return NotFound();
}
int pageSize = 3;
var episodeQuery = from e in _context.Episode select e;
episodeQuery = episodeQuery.Where(e => e.Movie.Id == id).OrderBy(e => e.Order);
var episodes = await PaginatedList<Episode>.CreateAsync(episodeQuery.AsNoTracking(), episodePage, pageSize);
return View(
new MovieDetailViewModel {
Movie = movie,
Episodes = episodes
});
}
public async Task<IActionResult> ByGenre(int id)
{
var genre = await _context.Genre
.Include(g => g.Movies)
.FirstOrDefaultAsync(g => g.Id == id);
if (genre == null)
{
return NotFound();
}
return View(genre);
}
}
}
| 544cb68975e768f26bf1976e41282d9922f8e91f | [
"C#"
] | 4 | C# | putuprema/beeflix-aspnet | 6b8e20180bdac613bb3252f17ad13ec5cfa3f16a | f1c4ed44d3ef104babf09154aca4ee237d3c9cbb | |
refs/heads/master | <repo_name>5uperN1na/hook-it-up<file_sep>/src/components/App.jsx
import React, { Fragment } from 'react';
import { BrowserRouter as Router, Link, Switch, Route } from 'react-router-dom';
import 'bootstrap/dist/css/bootstrap.min.css';
import Home from './Home';
import UserDetails from './UserDetails';
// import Navbar from './components/Navbar';
// import Card from 'react-bootstrap/Card';
// import ListGroup from 'react-bootstrap/ListGroup';
import 'isomorphic-fetch';
import 'es6-promise';
function App() {
// const [data, setData] = useState([]);
// useEffect(() => {
// loadData();
// }, []);
// const loadData = async () => {
// await fetch("https://jsonplaceholder.typicode.com/users")
// .then(response => response.json())
// .then(receivedData => setData(receivedData));
// }
// useState basic examples
// const [cartItems, setCartItems] = useState([]);
// useState example for scroll depth useEffect example below
// const [scrollDepth, setScrollDepth] = useState([0]);
// function determineUserScrollDepth() {
// const scrolled = document.documentElement.scrollTop ||
// document.body.scrollTop;
// setScrollDepth(scrolled);
// }
// useEffect(() => {
// window.addEventListener('scroll', determineUserScrollDepth);
// })
return (
<Router>
<Fragment>
<Switch>
<Route exact path="/" component={Home}></Route>
<Route exact path="/Users/:id" component={UserDetails} /> */}
<Route path="*" component={() => <h1 className="mt-5 text-center">404 Page</h1>} />
</Switch>
</Fragment>
</Router>
// <div className="App">
// {/* <Navbar /> */}
// <p> Fetch! </p>
// {data.map(users => (
// <div key = {users.id}>{users.name}</div>
// ))}
// </div>
// <div className="card">
// <p> Fetching Data! </p>
// {data.map(photos => (
// <ListGroup.Item key={photos.id}>
// <Card>
// <Card.Body><b>Blah:</b> {photos.title}</Card.Body>
// </Card>
// <div><br /></div>
// <b>Blah Blah:</b> {photos.url}
// </ListGroup.Item>
// ))};
// </div> */}
// {/* useState example below
// <p>
// Items in cart: {cartItems.toString()}
// </p>
// <button
// onClick={() =>
// setCartItems([...cartItems, 'apple'])
// }>
// Add item to Cart!
// </button> */}
// {/* Example of useEffect
// <p>You have scrolled down the page this far: {scrollDepth}</p>
// <p>
// Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
// Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
// Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
// Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
// Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
// Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
// Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
// Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
// Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Quis ipsum suspendisse ultrices gravida. A scelerisque purus semper eget. Nisi vitae suscipit tellus mauris. Nisl condimentum id venenatis a condimentum vitae. Dolor sit amet consectetur adipiscing elit. Rhoncus mattis rhoncus urna neque viverra justo nec. Vel elit scelerisque mauris pellentesque pulvinar pellentesque. Interdum varius sit amet mattis vulputate enim nulla aliquet porttitor. Placerat orci nulla pellentesque dignissim enim. At in tellus integer feugiat scelerisque. Volutpat ac tincidunt vitae semper quis. Tempor orci eu lobortis elementum nibh tellus molestie. Gravida cum sociis natoque penatibus et. Id venenatis a condimentum vitae sapien pellentesque habitant morbi.
// Egestas congue quisque egestas diam in arcu cursus euismod. Fusce ut placerat orci nulla pellentesque dignissim enim sit. Platea dictumst quisque sagittis purus. Ipsum suspendisse ultrices gravida dictum. Iaculis at erat pellentesque adipiscing commodo. Elementum sagittis vitae et leo. Eros in cursus turpis massa. Nisi porta lorem mollis aliquam ut. Fringilla urna porttitor rhoncus dolor. Vehicula ipsum a arcu cursus vitae congue mauris rhoncus aenean. Scelerisque mauris pellentesque pulvinar pellentesque habitant morbi. Fermentum et sollicitudin ac orci phasellus egestas tellus rutrum. In nisl nisi scelerisque eu ultrices vitae auctor eu. Lacus vestibulum sed arcu non odio euismod lacinia at quis. Velit sed ullamcorper morbi tincidunt ornare massa. Praesent elementum facilisis leo vel fringilla est ullamcorper eget.
// </p> */}
// // {/* </div > */}
);
}
export default App;<file_sep>/src/components/UserDetails.jsx
import React, { useState, useEffect, Fragment } from 'react';
import { BrowserRouter as Router, Link, Switch, Route } from 'react-router-dom';
import 'bootstrap/dist/css/bootstrap.min.css';
import Home from './Home';
// import Navbar from './components/Navbar';
//import { Card, CardText, CardTitle, CardBody } from 'react-bootstrap/Card';
// import ListGroup from 'react-bootstrap/ListGroup';
import 'isomorphic-fetch';
import 'es6-promise';
function UserDetails(props) {
// const details = {
// address: {
// street: ""
// }
// };
// const [data, setData] = useState([details]);
const [data, setData] = useState([]);
useEffect(() => {
loadData();
}, []);
const loadData = async () => {
await fetch("https://jsonplaceholder.typicode.com/users/" + props.match.params.id)
.then(response => response.json())
.then(receivedData => setData(receivedData));
}
return (
<div className="container">
<h1 className="text-align-right">Customer Details</h1>
<Fragment>
<div className="card" styles = {{width: '11rem'}}>
<div className="card-body">
<h4 className="card-title">{data.name}</h4>
<h5 className="text-align-right"><u>Address</u></h5>
Street: <h6 class="card-subtitle text-muted"> {data.address && <p>{data.address.street}</p>} </h6>
Suite: <h6 class="card-subtitle text-muted"> {data.address && <p>{data.address.suite}</p>}</h6>
City: <h6 class="card-subtitle text-muted"> {data.address && <p>{data.address.city}</p>}</h6>
Zip Code: <h6 class="card-subtitle text-muted"> {data.address && <p>{data.address.zipcode}</p>}</h6>
Lat: <h6 class="card-subtitle text-muted"> {data.address && data.address.geo && <p>{data.address.geo.lat}</p>}</h6>
Long: <h6 class="card-subtitle text-muted"> {data.address && data.address.geo && <p>{data.address.geo.lng}</p>}</h6>
<h5 className="text-align-right"><u>Phone</u></h5>
<h6 class="card-subtitle text-muted"> {data.phone}</h6>
<p></p>
<h5 className="text-align-right"><u>Website</u></h5>
< h6 class="card-subtitle text-muted"> {data.website}</h6>
<p></p>
<h5 className="text-align-right"><u>Company</u></h5>
<h6 class="card-subtitle text-muted"> {data.company && <p>{data.company.name}</p>}</h6>
<h6 class="card-subtitle text-muted"> {data.company && <p>{data.company.catchPhrase}</p>}</h6>
<h6 class="card-subtitle text-muted"> {data.company && <p>{data.company.bs}</p>}</h6>
</div>
</div>
</Fragment>
</div>
);
}
export default UserDetails; | cddb71c2d519ff303a3b1912d4bb87a12b4982e4 | [
"JavaScript"
] | 2 | JavaScript | 5uperN1na/hook-it-up | fea138f40dcc999b7b051ea4c78fa8163b82e5ae | cd6c924549af85649b73ed49fcd4e4d48b948f44 | |
refs/heads/master | <file_sep><?php
print system("cat /etc/passwd");
?> | 9248ae302068b6159309acf08a4cf83583fa4d05 | [
"PHP"
] | 1 | PHP | martinezlinux/projetos | d5d1147b37a1443aa440bd9341bdb44739691100 | 0a45771ef5d274b69ee6303786d8cb46f79932e6 | |
refs/heads/main | <repo_name>stefanociccalotti/linear-problems<file_sep>/src/vectorSpace/euclidean/EuclideanVector.java
package vectorSpace.euclidean;
import vectorSpace.Vector;
public class EuclideanVector implements Vector{
private int components[];
private int dimension;
public EuclideanVector() {}
public EuclideanVector(int dimension) {
this.components = new int[dimension];
this.dimension = dimension;
}
public EuclideanVector(int[] components) {
this.components = components;
this.dimension = components.length;
}
public int[] getComponents() {
return this.components;
}
public int getAxisComponent(int axis) {
return this.components[axis];
}
public int getDimension() {
return this.dimension;
}
public void setComponents(int[] components) {
this.components = components;
}
public void setAxisComponent(int axis, int component) {
this.components[axis] = component;
}
public void copyVector(Vector v) {
int[] components = new int[v.getDimension()];
int[] tempComponents = v.getComponents();
for(int i = 0; i < components.length; i++) {
components[i] = tempComponents[i];
}
this.components = components;
}
public String toString() {
String s = "(";
int i;
for(i = 0; i < components.length-1; i++) {
s+=components[i]+", ";
}
s+=components[i]+")\n";
return s;
}
}
<file_sep>/src/LinearCombination.java
import vectorSpace.*;
import vectorSpace.euclidean.*;
public class LinearCombination {
public static void main(String[] args) {
//wtf
Vector[] base = new EuclideanVector[]{ new EuclideanVector(new int[]{0, 0, 1}),
new EuclideanVector(new int[]{0, 1, 0}),
new EuclideanVector(new int[]{1, 0, 0})
};
Space space = new EuclideanSpaceCanonicalBase(base);
Vector v1 = new EuclideanVector(new int[]{1, 2, 3});
Vector v2 = new EuclideanVector(new int[]{1, 6, 2});
space.associateVector(v1);
space.associateVector(v2);
Vector v3 = new EuclideanVector();
v3.copyVector(v1);
EuclideanSpaceCanonicalBase.scaleEuclideanVector(v3, 2);
EuclideanSpaceCanonicalBase.addEuclideanVectors(v3, v2);
space.associateVector(v3);
space.printSpaceAndVectors();
}
}
| bca424868a566812817b5fa2878d85f4248e0894 | [
"Java"
] | 2 | Java | stefanociccalotti/linear-problems | 0282e60d5738e9a26c5be2d63f49696ae2e08a40 | 2f96eef2aff7076e488560b1e6c343c0ea533d87 | |
refs/heads/master | <repo_name>Maudulus/car_listing<file_sep>/app/models/car.rb
class Car < ActiveRecord::Base
belongs_to :manufacturer
validates :color, presence: true
validates :year, presence: true,
numericality: { greater_than_or_equal_to: 1979, less_than_or_equal_to: Date.current.year }
validates :mileage, presence: true, numericality: true
end
<file_sep>/spec/models/manufacturer_spec.rb
require 'spec_helper'
describe Manufacturer do
it { should have_valid(:name).when('Toyota') }
it { should_not have_valid(:name).when(nil, '') }
it { should have_valid(:country).when('Africa') }
it { should_not have_valid(:country).when(nil, '') }
it { should have_many(:cars).dependent(:nullify) }
end
<file_sep>/spec/support/factories.rb
FactoryGirl.define do
factory :car do
color 'green'
year '1980'
mileage '200000'
description 'Not only does this car look sporty and powerful, it also has aerodynamics equivalent to a garden shed, enabling it to reach speeds well beyond 100,000 metres per hour. In fact, with a tail wind these things can go even faster, but a cross wind presents problems, especially after the car ends up on its roof.'
end
factory :manufacturer do
name '<NAME>'
end
end
<file_sep>/spec/features/user_adds_manufacturer_spec.rb
require 'spec_helper'
feature 'used car salesperson specifies manufacturer (optionally)', %q{
As a car salesperson
I want to record a car manufacturer
So that I can keep track of the types of cars found in the lot
} do
scenario 'salesperson adds a car with valid attributes' do
manufacturer = FactoryGirl.build(:manufacturer)
count = Manufacturer.count
visit new_manufacturer_path
new_manufacturer_helper(manufacturer)
select('United Kingdom', from: 'manufacturer_country')
click_on 'Add Manufacturer'
expect(Manufacturer.count).to eq(count+1)
expect(page).to have_content("Manufacturer Successfully Added")
expect(current_path).to eq new_manufacturer_path
end
scenario 'salesperson adds a car with invalid attributes' do
manufacturer = FactoryGirl.build(:manufacturer)
count = Manufacturer.count
visit new_manufacturer_path
new_manufacturer_helper(manufacturer)
click_on 'Add Manufacturer'
expect(Manufacturer.count).to eq(count)
expect(page).to have_content("Manufacturer Not Added")
expect(current_path).to eq manufacturers_path
end
end
<file_sep>/spec/features/user_adds_car_spec.rb
require 'spec_helper'
feature 'used car salesperson adds car', %q{
As a car salesperson
I want to record a newly acquired car
So that I can list it in my lot
} do
scenario 'salesperson adds a car with valid attributes' do
car = FactoryGirl.build(:car)
count = Car.count
visit new_car_path
new_car_helper(car)
click_on 'Add Car'
expect(Car.count).to eq(count+1)
expect(page).to have_content("Car Successfully Added")
expect(current_path).to eq new_car_path
end
scenario 'salesperson adds a car with invalid attributes' do
car = FactoryGirl.build(:car)
count = Car.count
visit new_car_path
new_car_helper(car)
fill_in "Color", with: ""
click_on 'Add Car'
expect(Car.count).to eq(count)
expect(page).to have_content("Car Not Added")
expect(current_path).to eq cars_path
end
end
<file_sep>/spec/models/car_spec.rb
require 'spec_helper'
describe Car do
it { should have_valid(:color).when('turquoise') }
it { should_not have_valid(:color).when(nil, '') }
it { should have_valid(:year).when('1980', '2014') }
it { should_not have_valid(:year).when(nil, '', 'Nineteen Eighty-Four', 1977, 2015) }
it { should have_valid(:mileage).when('200000') }
it { should_not have_valid(:mileage).when(nil, '', "asdf") }
it { should belong_to :manufacturer }
end
<file_sep>/spec/support/form_input_helper.rb
module FormHelper
def new_car_helper(valid_attrs)
fill_in 'Color', with: valid_attrs.color
fill_in 'Year', with: valid_attrs.year
fill_in 'Mileage', with: valid_attrs.mileage
fill_in 'Description', with: valid_attrs.description
end
def new_manufacturer_helper(valid_attrs)
fill_in 'Name', with: valid_attrs.name
end
end
| da86e8aa2ed0ec81f4d1f47d5e2b8ae3799056fb | [
"Ruby"
] | 7 | Ruby | Maudulus/car_listing | 89457999c566635345d5a555f9a4ceca8471adb2 | 0a8736447fde87fee801fcb87ecc5965041e954f | |
refs/heads/master | <file_sep>var wppt_chart;
var wppt_result = {
r: 0,
i: 0,
a: 0,
s: 0,
e: 0,
c: 0
};
var wppt_score = {
r: 0,
i: 0,
a: 0,
s: 0,
e: 0,
c: 0
};
// functions
var wppt_content, wppt_report, wppt_questionnaire_done, wppt_display_score;
jQuery(document).ready(function($){
wppt_chart = {
bar1: $('.graph-container > li:nth-child(1) .bar-inner'),
bar2: $('.graph-container > li:nth-child(2) .bar-inner'),
bar3: $('.graph-container > li:nth-child(3) .bar-inner'),
bar4: $('.graph-container > li:nth-child(4) .bar-inner'),
bar5: $('.graph-container > li:nth-child(5) .bar-inner'),
bar6: $('.graph-container > li:nth-child(6) .bar-inner')
};
$('.wppt-start').click(function(e){
e.preventDefault();
Avgrund.show('#acquire-user-data');
$('html, body').animate({ scrollTop: (($(document).height() / 2) - ($('#acquire-user-data').height())) }, 500);
})
$('.close-popup').live('click', function(e){
e.preventDefault();
// check for user data validity
if($('.userdata-name').val().length == 0){
alert('Please enter your name!');
$('.userdata-name').focus();
return;
}
// store user data
wppt_data.name = $('.userdata-name').val();
$('.report-userdata-name').text(wppt_data.name);
$('.content-userdata-name').text(wppt_data.name);
Avgrund.hide();
wppt_content();
});
$('.wppt-finish').click(function(e){
e.preventDefault();
if(!wppt_questionnaire_done()){
alert('Please complete the questionnaire!');
return;
}
wppt_report();
});
wppt_questionnaire_done = function(){
for (var i = $('#qa-list .qa-item').length - 1; i >= 0; i--) {
if(!$('input[type="radio"]:checked', $('#qa-list .qa-item')[i]).length){
return false;
}
};
return true;
}
var reset_chart = function(){
wppt_chart.bar1.css({'height': 0, 'bottom': '-2.5em'});
wppt_chart.bar2.css({'height': 0, 'bottom': '-2.5em'});
wppt_chart.bar3.css({'height': 0, 'bottom': '-2.5em'});
wppt_chart.bar4.css({'height': 0, 'bottom': '-2.5em'});
wppt_chart.bar5.css({'height': 0, 'bottom': '-2.5em'});
wppt_chart.bar6.css({'height': 0, 'bottom': '-2.5em'});
}
wppt_content = function(){
$('#wppt-intro').removeClass('show-step').addClass('hide-step');
$('#wppt-content').removeClass('hide-step').addClass('show-step');
$('html, body').animate({ scrollTop: $("#wppt-content").offset().top - 80 }, 500);
}
wppt_display_score = function(){
wppt_chart.bar1.css({'height': wppt_score.r + '%', 'bottom': (wppt_score.r == 0?'-2.5em':0)});
wppt_chart.bar2.css({'height': wppt_score.i + '%', 'bottom': (wppt_score.i == 0?'-2.5em':0)});
wppt_chart.bar3.css({'height': wppt_score.a + '%', 'bottom': (wppt_score.a == 0?'-2.5em':0)});
wppt_chart.bar4.css({'height': wppt_score.s + '%', 'bottom': (wppt_score.s == 0?'-2.5em':0)});
wppt_chart.bar5.css({'height': wppt_score.e + '%', 'bottom': (wppt_score.e == 0?'-2.5em':0)});
wppt_chart.bar6.css({'height': wppt_score.c + '%', 'bottom': (wppt_score.c == 0?'-2.5em':0)});
}
wppt_report = function(){
// calculate result
var nqa = $('#qa-list .qa-item').length;
for(component in wppt_result){
wppt_result[component] = $('#qa-list .qa-item input[value="' + component + '"]:checked').length;
}
// setup chart
reset_chart();
wppt_score = {
r: ((wppt_result.r > 0)?(Math.floor(wppt_result.r / nqa * 100)):0),
i: ((wppt_result.i > 0)?(Math.floor(wppt_result.i / nqa * 100)):0),
a: ((wppt_result.a > 0)?(Math.floor(wppt_result.a / nqa * 100)):0),
s: ((wppt_result.s > 0)?(Math.floor(wppt_result.s / nqa * 100)):0),
e: ((wppt_result.e > 0)?(Math.floor(wppt_result.e / nqa * 100)):0),
c: ((wppt_result.c > 0)?(Math.floor(wppt_result.c / nqa * 100)):0)
};
// presentate result value
$('.graph-container > li:nth-child(1) .component-result').text( wppt_score.r + '%');
$('.graph-container > li:nth-child(2) .component-result').text( wppt_score.i + '%');
$('.graph-container > li:nth-child(3) .component-result').text( wppt_score.a + '%');
$('.graph-container > li:nth-child(4) .component-result').text( wppt_score.s + '%');
$('.graph-container > li:nth-child(5) .component-result').text( wppt_score.e + '%');
$('.graph-container > li:nth-child(6) .component-result').text( wppt_score.c + '%');
// disable inputs
$('#qa-list .qa-item').css({'color': 'gray'});
$('#qa-list .qa-item input').attr('disabled','disabled');
$('#wppt-report').removeClass('hide-step').removeClass('hidden').addClass('show-step');
$('html, body').animate({ scrollTop: ($("#wppt-report").offset().top - 40) }, 500);
setTimeout('wppt_display_score();', 1500);
}
});<file_sep>### Personality Test Plugin For Wordpress
This intent to provide personality test wit these elements involved:
**(RIASEC)**
1. **R**ealistic
2. **I**nvestigative
3. **A**rtistic
4. **S**ocial
5. **E**nterprising
6. **C**onvensional
You can read more about each elements [here](http://www.entrepreneurrumahan.com/kuesioner-minat/cara-membaca-hasil/) (it's in Indonesian), for english explaination you can read it [here](http://sourcesofinsight.com/6-personality-and-work-environment-types/)
#### Installing
1. Drop the **wppt** folder into plugins/


2. **Activate** the plugin
#### Backend (Plugin | wp-admin)
**Menu**

**Setting**




**The Test Listing**

#### Using The Shorcode
The shortcode is straight-forward, just place it in any Page or Post, it will render the related questions.
#### Interface
**Intro**


**Questions**

**Report**

***
This plugin is installed at : http://www.entrepreneurrumahan.com/kuesioner-minat/ | 1b64a4086e077a0d62972b7f58fb5d2cf8bcb053 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | lvvvvvl/wp-personality-test | c4fe01b1780fffe1c769b02a90262b7711a4cf3d | 998aa60f92f4a43e38745e9828a3b7b491405c39 | |
refs/heads/master | <file_sep>
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data.Entity;
using STAWebsite2.Models;
namespace STAWebsite2.DAL
{
public class STAInitializer : System.Data.Entity.DropCreateDatabaseIfModelChanges<STAContext>
{
protected override void Seed(STAContext context)
{
var students = new List<Student>
{
new Student{Name="<NAME>",StudentID=160104001,Semester=2,Year=3,WarningNo=0,LoginStatus="yes",Password="<PASSWORD>"},
new Student{Name="<NAME>",StudentID=160104008,Semester=2,Year=3,WarningNo=0,LoginStatus="yes",Password="<PASSWORD>"},
new Student{Name="<NAME>",StudentID=160104012,Semester=2,Year=3,WarningNo=0,LoginStatus="yes",Password="<PASSWORD>"}
};
students.ForEach(s => context.Students.Add(s));
context.SaveChanges();
var courses = new List<Course>
{
new Course{CourseID=3211,Title="Data Communication",avgSCRating=0,verificationCode="hf4ry7"},
new Course{CourseID=3213,Title="Operating System",avgSCRating=0,verificationCode="vfd34x"},
new Course{CourseID=3214,Title="Operating System Lab",avgSCRating=0,verificationCode="45lshf"},
new Course{CourseID=3215,Title="Microcontroller based System Design",avgSCRating=0,verificationCode="hdf12d"},
new Course{CourseID=3216,Title="Microcontroller based System Design Lab",avgSCRating=0,verificationCode="as5bhg"},
new Course{CourseID=3223,Title="Information System Design & Software Engineering",avgSCRating=0,verificationCode="7tet89"},
new Course{CourseID=3224,Title="Information System Design & Software Engineering Lab",avgSCRating=0,verificationCode="gh457r"},
new Course{CourseID=3200,Title="Software Development V",avgSCRating=0,verificationCode="h8t5uh"},
new Course{CourseID=3207,Title="Industrial Law & Safety Management",avgSCRating=0,verificationCode="s030ii"}
};
courses.ForEach(s => context.Courses.Add(s));
context.SaveChanges();
var instructors = new List<Instructor>
{
new Instructor{InstructorID=0001,Name="<NAME>",avgIRating=0,Department="CSE",LoginStatus="no",Password="<PASSWORD>",WarningNo=0},
new Instructor{InstructorID=0002,Name="<NAME>",avgIRating=0,Department="CSE",LoginStatus="no",Password="<PASSWORD>",WarningNo=0},
new Instructor{InstructorID=0003,Name="<NAME>",avgIRating=0,Department="CSE",LoginStatus="no",Password="<PASSWORD>",WarningNo=0}
};
instructors.ForEach(s => context.Instructors.Add(s));
context.SaveChanges();
var iratings = new List<IRating>
{
new IRating{SubCriteria1=5,SubCriteria2=5,SubCriteria3=5,SubCriteria4=5,SubCriteria5=5,SubCriteria6=5,
SubCriteria7 =5,SubCriteria8=5,SubCriteria9=5,SubCriteria10=5,SubCriteria11=5,SubCriteria12=5,
SubCriteria13 =5,SubCriteria14=5,SubCriteria15=5,SubCriteria16=5,SubCriteria17=5,SubCriteria18=5,
SubCriteria19 =5,SubCriteria20=5,Review="Today's class was very englightening.",StudentID=160104001,InstructorID=0001}
};
iratings.ForEach(s => context.IRatings.Add(s));
context.SaveChanges();
var scratings = new List<SCRating>
{
new SCRating{InstructorID=0001,CourseID=3213,SubCriteria1=5,SubCriteria2=5,SubCriteria3=5,SubCriteria4=5,SubCriteria5=5,SubCriteria6=5,SubCriteria7=5}
};
scratings.ForEach(s => context.SCRatings.Add(s));
context.SaveChanges();
var studentcourses = new List<Student_Course>
{
new Student_Course{CourseID=3213,StudentID=160104001},
new Student_Course{CourseID=3214,StudentID=160104001},
new Student_Course{CourseID=3213,StudentID=160104008}
};
studentcourses.ForEach(s => context.Student_Courses.Add(s));
context.SaveChanges();
}
}
}<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Web;
namespace STAWebsite2.Models
{
public class Course
{
[DatabaseGenerated(DatabaseGeneratedOption.None)]
public int CourseID { get; set; }
public string Title { get; set; }
public float avgSCRating { get; set; }
public string verificationCode { get; set; }
public int? InstructorID { get; set; }
public virtual Instructor Instructor { get; set; }
public virtual ICollection<Student_Course> Student_Courses { get; set; }
public virtual ICollection<SCRating> SCRatings { get; set; }
}
}<file_sep># CSE3200-Software-Development-V<file_sep>using STAWebsite2.Models;
using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Data.Entity.ModelConfiguration.Conventions;
using System.Linq;
using System.Web;
namespace STAWebsite2.DAL
{
public class STAContext :DbContext
{
public STAContext() : base("STAContext")
{
}
public DbSet<Student> Students { get; set; }
public DbSet<Instructor> Instructors { get; set; }
public DbSet<Course> Courses { get; set; }
public DbSet<SCRating> SCRatings { get; set; }
public DbSet<IRating> IRatings { get; set; }
public DbSet<Student_Course> Student_Courses { get; set; }
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
modelBuilder.Conventions.Remove<PluralizingTableNameConvention>();
}
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace STAWebsite2.Models
{
public class Student_Course
{
public int Student_CourseID { get; set; }
public int? CourseID { get; set; }
public int? StudentID { get; set; }
public virtual Student Student { get; set; }
public virtual Course Course { get; set; }
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace STAWebsite2.Models
{
public class IRating
{
public int IRatingID { get; set; }
public int SubCriteria1 { get; set; }
public int SubCriteria2 { get; set; }
public int SubCriteria3 { get; set; }
public int SubCriteria4 { get; set; }
public int SubCriteria5 { get; set; }
public int SubCriteria6 { get; set; }
public int SubCriteria7 { get; set; }
public int SubCriteria8 { get; set; }
public int SubCriteria9 { get; set; }
public int SubCriteria10 { get; set; }
public int SubCriteria11 { get; set; }
public int SubCriteria12 { get; set; }
public int SubCriteria13 { get; set; }
public int SubCriteria14 { get; set; }
public int SubCriteria15 { get; set; }
public int SubCriteria16 { get; set; }
public int SubCriteria17 { get; set; }
public int SubCriteria18 { get; set; }
public int SubCriteria19 { get; set; }
public int SubCriteria20 { get; set; }
public string Review { get; set; }
public virtual Student Student { get; set; }
public virtual Instructor Instructor { get; set; }
public int? StudentID { get; set; }
public int? InstructorID { get; set; }
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.Linq;
using System.Net;
using System.Web;
using System.Web.Mvc;
using STAWebsite2.DAL;
using STAWebsite2.Models;
namespace STAWebsite2.Controllers
{
public class IRatingController : Controller
{
private STAContext db = new STAContext();
// GET: IRating
public ActionResult Index()
{
var iRatings = db.IRatings.Include(i => i.Instructor).Include(i => i.Student);
return View(iRatings.ToList());
}
// GET: IRating/Details/5
public ActionResult Details(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
IRating iRating = db.IRatings.Find(id);
if (iRating == null)
{
return HttpNotFound();
}
return View(iRating);
}
// GET: IRating/Create
public ActionResult Create()
{
ViewBag.InstructorID = new SelectList(db.Instructors, "InstructorID", "Name");
ViewBag.StudentID = new SelectList(db.Students, "StudentID", "Name");
return View();
}
// POST: IRating/Create
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see https://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create([Bind(Include = "IRatingID,SubCriteria1,SubCriteria2,SubCriteria3,SubCriteria4,SubCriteria5,SubCriteria6,SubCriteria7,SubCriteria8,SubCriteria9,SubCriteria10,SubCriteria11,SubCriteria12,SubCriteria13,SubCriteria14,SubCriteria15,SubCriteria16,SubCriteria17,SubCriteria18,SubCriteria19,SubCriteria20,Review,StudentID,InstructorID")] IRating iRating)
{
if (ModelState.IsValid)
{
db.IRatings.Add(iRating);
db.SaveChanges();
return RedirectToAction("Index");
}
ViewBag.InstructorID = new SelectList(db.Instructors, "InstructorID", "Name", iRating.InstructorID);
ViewBag.StudentID = new SelectList(db.Students, "StudentID", "Name", iRating.StudentID);
return View(iRating);
}
// GET: IRating/Edit/5
public ActionResult Edit(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
IRating iRating = db.IRatings.Find(id);
if (iRating == null)
{
return HttpNotFound();
}
ViewBag.InstructorID = new SelectList(db.Instructors, "InstructorID", "Name", iRating.InstructorID);
ViewBag.StudentID = new SelectList(db.Students, "StudentID", "Name", iRating.StudentID);
return View(iRating);
}
// POST: IRating/Edit/5
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see https://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Edit([Bind(Include = "IRatingID,SubCriteria1,SubCriteria2,SubCriteria3,SubCriteria4,SubCriteria5,SubCriteria6,SubCriteria7,SubCriteria8,SubCriteria9,SubCriteria10,SubCriteria11,SubCriteria12,SubCriteria13,SubCriteria14,SubCriteria15,SubCriteria16,SubCriteria17,SubCriteria18,SubCriteria19,SubCriteria20,Review,StudentID,InstructorID")] IRating iRating)
{
if (ModelState.IsValid)
{
db.Entry(iRating).State = EntityState.Modified;
db.SaveChanges();
return RedirectToAction("Index");
}
ViewBag.InstructorID = new SelectList(db.Instructors, "InstructorID", "Name", iRating.InstructorID);
ViewBag.StudentID = new SelectList(db.Students, "StudentID", "Name", iRating.StudentID);
return View(iRating);
}
// GET: IRating/Delete/5
public ActionResult Delete(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
IRating iRating = db.IRatings.Find(id);
if (iRating == null)
{
return HttpNotFound();
}
return View(iRating);
}
// POST: IRating/Delete/5
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public ActionResult DeleteConfirmed(int id)
{
IRating iRating = db.IRatings.Find(id);
db.IRatings.Remove(iRating);
db.SaveChanges();
return RedirectToAction("Index");
}
protected override void Dispose(bool disposing)
{
if (disposing)
{
db.Dispose();
}
base.Dispose(disposing);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace STAWebsite2.Models
{
public class SCRating
{
public int SCRatingID { get; set; }
public int SubCriteria1 { get; set; }
public int SubCriteria2 { get; set; }
public int SubCriteria3 { get; set; }
public int SubCriteria4 { get; set; }
public int SubCriteria5 { get; set; }
public int SubCriteria6 { get; set; }
public int SubCriteria7 { get; set; }
public string Review { get; set; }
public virtual Instructor Instructor { get; set; }
public virtual Course Course { get; set; }
public int? CourseID { get; set; }
public int? InstructorID { get; set; }
}
}<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Web;
namespace STAWebsite2.Models
{
public class Instructor
{
[DatabaseGenerated(DatabaseGeneratedOption.None)]
public int InstructorID { get; set; }
[Required(ErrorMessage = "*Enter valid name")]
[Display(Name = "Instructor Name")]
public string Name { get; set; }
public string Department { get; set; }
[Required(ErrorMessage = "*Enter valid password")]
[DataType(DataType.Password)]
[Display(Name = "Password")]
public string Password { get; set; }
public float avgIRating { get; set; }
public int WarningNo { get; set; }
public string LoginStatus { get; set; }
public virtual ICollection<Course> Courses { get; set; }
public virtual ICollection<IRating> IRatings { get; set; }
public virtual ICollection<SCRating> SCRatings { get; set; }
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.Linq;
using System.Net;
using System.Web;
using System.Web.Mvc;
using STAWebsite2.DAL;
using STAWebsite2.Models;
namespace STAWebsite2.Controllers
{
public class SCRatingController : Controller
{
private STAContext db = new STAContext();
// GET: SCRating
public ActionResult Index()
{
var sCRatings = db.SCRatings.Include(s => s.Course).Include(s => s.Instructor);
return View(sCRatings.ToList());
}
// GET: SCRating/Details/5
public ActionResult Details(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
SCRating sCRating = db.SCRatings.Find(id);
if (sCRating == null)
{
return HttpNotFound();
}
return View(sCRating);
}
// GET: SCRating/Create
public ActionResult Create()
{
ViewBag.CourseID = new SelectList(db.Courses, "CourseID", "Title");
ViewBag.InstructorID = new SelectList(db.Instructors, "InstructorID", "Name");
return View();
}
// POST: SCRating/Create
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see https://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create([Bind(Include = "SCRatingID,SubCriteria1,SubCriteria2,SubCriteria3,SubCriteria4,SubCriteria5,SubCriteria6,SubCriteria7,Review,CourseID,InstructorID")] SCRating sCRating)
{
if (ModelState.IsValid)
{
db.SCRatings.Add(sCRating);
db.SaveChanges();
return RedirectToAction("Index");
}
ViewBag.CourseID = new SelectList(db.Courses, "CourseID", "Title", sCRating.CourseID);
ViewBag.InstructorID = new SelectList(db.Instructors, "InstructorID", "Name", sCRating.InstructorID);
return View(sCRating);
}
// GET: SCRating/Edit/5
public ActionResult Edit(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
SCRating sCRating = db.SCRatings.Find(id);
if (sCRating == null)
{
return HttpNotFound();
}
ViewBag.CourseID = new SelectList(db.Courses, "CourseID", "Title", sCRating.CourseID);
ViewBag.InstructorID = new SelectList(db.Instructors, "InstructorID", "Name", sCRating.InstructorID);
return View(sCRating);
}
// POST: SCRating/Edit/5
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see https://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Edit([Bind(Include = "SCRatingID,SubCriteria1,SubCriteria2,SubCriteria3,SubCriteria4,SubCriteria5,SubCriteria6,SubCriteria7,Review,CourseID,InstructorID")] SCRating sCRating)
{
if (ModelState.IsValid)
{
db.Entry(sCRating).State = EntityState.Modified;
db.SaveChanges();
return RedirectToAction("Index");
}
ViewBag.CourseID = new SelectList(db.Courses, "CourseID", "Title", sCRating.CourseID);
ViewBag.InstructorID = new SelectList(db.Instructors, "InstructorID", "Name", sCRating.InstructorID);
return View(sCRating);
}
// GET: SCRating/Delete/5
public ActionResult Delete(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
SCRating sCRating = db.SCRatings.Find(id);
if (sCRating == null)
{
return HttpNotFound();
}
return View(sCRating);
}
// POST: SCRating/Delete/5
[HttpPost, ActionName("Delete")]
[ValidateAntiForgeryToken]
public ActionResult DeleteConfirmed(int id)
{
SCRating sCRating = db.SCRatings.Find(id);
db.SCRatings.Remove(sCRating);
db.SaveChanges();
return RedirectToAction("Index");
}
protected override void Dispose(bool disposing)
{
if (disposing)
{
db.Dispose();
}
base.Dispose(disposing);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
namespace STAWebsite2.Models
{
public class Student
{
[DatabaseGenerated(DatabaseGeneratedOption.None)]
public int StudentID { get; set; }
[Required(ErrorMessage = "*Enter valid name")]
[Display(Name = "Student Name")]
public string Name { get; set; }
public int Year { get; set; }
public int Semester { get; set; }
[Required(ErrorMessage = "*Enter valid password")]
[DataType(DataType.Password)]
[Display(Name = "Password")]
public string Password { get; set; }
public int WarningNo { get; set; }
public string LoginStatus { get; set; }
public virtual ICollection<Student_Course> Student_Courses { get; set; }
public virtual ICollection<IRating> IRatings { get; set; }
}
} | e2ba14fdd1b74ff26cd43115a16cd54ece6b4aaf | [
"Markdown",
"C#"
] | 11 | C# | tajbiahossain/Student-Teacher-Assessment-Website | a3eca2314912ba2ca9d31efef5fdff8ee67302c4 | c3d0d69bf6bc0af60b48c47a7e8940d5c428e653 | |
refs/heads/master | <repo_name>YSOUZAS/javascript-course-from-standards-to-a-functional-approach<file_sep>/public/app/console-handler.js
import { EventEmitter } from './utils/event-emitter.js'
EventEmitter.on('itensTotalizados', console.log);
<file_sep>/README.md
# JavaScript Course: From standards to a functional approach
- [Curso JavaScript: De padrões a uma abordagem funcional](https://cursos.alura.com.br/course/javascript-padroes-abordagem-funcional)
- run project
- `npm install`
- `node serve.js`
| 90b57ea94c280a55522d6dae8df6b58eb8fe436b | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | YSOUZAS/javascript-course-from-standards-to-a-functional-approach | 2769f5c6cccc91290d8147a170bf16b4a21adc71 | 8efb4df92386484f682538e4cabb91d8e272a39a | |
refs/heads/master | <file_sep>HalfImageSize
=============
## HalfImageSizeについて
Sublime Text2用のプラグインです。
img要素のwidth, heightの値を半分にします。
スマホサイトを作る場合に便利な痒いところに手が届くプラグインとなっています。
## 使い方
以下の様なimg要素に対して
```html
<img src="hoge.jpg" width="100" height="100" />
```
img要素を選択した後、<kbd>ctrl</kbd>+<kbd>alt</kbd>+<kbd>h</kbd>を押すことで
```html
<img src="hoge.jpg" width="50" height="50" />
```
とwidth, heightの値が半分になります。
因みに要素を選択しなくても<kbd>ctrl</kbd>+<kbd>shift</kbd>+<kbd>h</kbd>を押すことで
現在の**行**に対してwidth, heightの値を半分にすることができます。
また、<kbd>ctrl</kbd>+<kbd>alt</kbd>+<kbd>shift</kbd>+<kbd>h</kbd>を押すことで
現在の**ファイル**に対してwidth, heightの値を半分にします。
<file_sep>import sublime, sublime_plugin, re, math
wh_matcher = re.compile(r'(width|height)=\"(\d*)\"');
def halveWidthHeightNum(matchobj):
halved_num = math.ceil(int(matchobj.group(2), 10) / 2)
halved_string = '%s="%d"' % (matchobj.group(1), halved_num)
return halved_string
def moveCaretPosition(self, point):
self.view.sel().clear()
self.view.sel().add(sublime.Region(point))
class HalfImageSizeCommand(sublime_plugin.TextCommand):
def run(self, edit):
selected_region = self.view.sel()[0]
selected_string = self.view.substr(selected_region)
halved_string = wh_matcher.sub(halveWidthHeightNum, selected_string)
self.view.replace(edit, selected_region, halved_string)
class HalfImageSizeLineCommand(sublime_plugin.TextCommand):
def run(self, edit):
# get current caret position
current_caret_position = self.view.sel()[0].begin()
current_line_begin_point = self.view.line(current_caret_position).begin()
current_line_end_point = self.view.line(current_caret_position).end()
# selected current line charactors
current_line_region = sublime.Region(current_line_begin_point, current_line_end_point)
current_line_string = self.view.substr(current_line_region)
halved_string = wh_matcher.sub(halveWidthHeightNum, current_line_string)
self.view.replace(edit, current_line_region, halved_string)
moveCaretPosition(self, current_caret_position)
class HalfImageSizeAllCommand(sublime_plugin.TextCommand):
def run(self, edit):
# get current caret position
# selected all charactors
all_region = sublime.Region(0, self.view.size())
selected_string = self.view.substr(all_region)
current_caret_position = self.view.sel()[0].begin()
halved_string = wh_matcher.sub(halveWidthHeightNum, selected_string)
self.view.replace(edit, all_region, halved_string)
moveCaretPosition(self, current_caret_position)
| f20687fd7265af223867bb623a249a6cf9e8be49 | [
"Markdown",
"Python"
] | 2 | Markdown | Satoh-D/HalfImageSize | dd8985edb9d0097362fda37431b7a3eea23953e0 | c354475b268daccddb176713912eda024e36220a | |
refs/heads/master | <repo_name>MTKGroup/LookAndFeel<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/LightButton.cs
using LookAndFeel.Components;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Timers;
using System.Threading;
namespace LookAndFeel.Controls
{
/**
*
*/
public class LightButton : Button
{
System.Timers.Timer timer = new System.Timers.Timer();
private DateTime backupTime;
System.Threading.Thread thread;
private double pressedTime = 0;
private double TotalTime = 700;
private bool isWorking = false;
/**
*
*/
public LightButton()
{
this.BackColor = Color.White;
this.ForeColor = Color.FromArgb(150, 40, 27);
this.Font = new Font("Segoe UI", 8);
}
public LightButton(ComponentInfo info)
: base(info)
{
this.BackColor = Color.White;
this.ForeColor = Color.FromArgb(150, 40, 27);
this.Font = new Font("Segoe UI", 8);
SetStyle(System.Windows.Forms.ControlStyles.StandardClick | System.Windows.Forms.ControlStyles.StandardDoubleClick, true);
thread = new System.Threading.Thread(
() => {
abc:
if (!isWorking) goto abc;
var distance = (DateTime.Now - backupTime).TotalMilliseconds;
if (distance >= 1000 / 60 &&
MouseButtons != System.Windows.Forms.MouseButtons.None
&& pressedTime < TotalTime)
{
pressedTime += distance;
Invalidate();
backupTime = DateTime.Now;
}
else if (pressedTime >= TotalTime)
{
isWorking = false;
this.Invoke((System.Windows.Forms.MethodInvoker)(()=>{
foreach (var h in clickHandlers)
{
h(this);
}
}));
}
goto abc;
}, 0);
thread.Start();
}
public override IComponent clone(ComponentInfo info)
{
var newControl = new LightButton(info);
//set info for newControl
//....
return newControl;
}
protected override void OnMouseDown(System.Windows.Forms.MouseEventArgs e)
{
base.OnMouseDown(e);
backupTime = DateTime.Now;
pressedTime = 0;
isWorking = true;
}
protected override void OnMouseUp(System.Windows.Forms.MouseEventArgs e)
{
base.OnMouseUp(e);
isWorking = false;
pressedTime = 0;
Invalidate();
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
//Draw border
//Brush b = new SolidBrush(this.BackColor);
//e.Graphics.DrawRectangle(new Pen(Color.FromArgb(51, 110, 123), 2), 0, 0, this.Width, this.Height);
try
{
base.OnPaintBackground(e);
Color backColor = BackColor;
Color foreColor = ForeColor;
Color borderColor = ForeColor;
if (isHovered && !isPressed && Enabled)
{
backColor = Color.FromArgb(255, 246, 237);
}
else if (isHovered && isPressed && Enabled)
{
//backColor = BackColor;
}
else if (Focused)
{
foreColor = Color.FromArgb(
Math.Min((int)(ForeColor.R * 1.4f), 255),
Math.Min((int)(ForeColor.G * 1.4f), 255),
Math.Min((int)(ForeColor.B * 1.4f), 255));
}
if (backColor.A == 255 && BackgroundImage == null)
{
e.Graphics.Clear(backColor);
}
//Draw percent
if (MouseButtons != System.Windows.Forms.MouseButtons.None && pressedTime > 0)
{
backColor = Color.FromArgb(255, 246, 237);
var percentColor = Color.FromArgb(
(int)(backColor.R * .7f),
(int)(backColor.G * .7f),
(int)(backColor.B * .7f));
percentColor = Color.FromArgb(255 , 146, 142);
e.Graphics.FillRectangle(new SolidBrush(percentColor), 0, 0,
(float)this.pressedTime / (float)this.TotalTime * (this.Width - 1),
this.Height);
}
e.Graphics.DrawRectangle(new Pen(borderColor), 0, 0, this.Width - 1, this.Height - 1);
System.Windows.Forms.TextRenderer.DrawText(e.Graphics,
this.Text, this.Font,
new Point(this.Width, this.Height / 2),
foreColor,
Color.Transparent,
System.Windows.Forms.TextFormatFlags.HorizontalCenter | System.Windows.Forms.TextFormatFlags.VerticalCenter);
}
catch
{
Invalidate();
}
}
protected override void OnPaintBackground(System.Windows.Forms.PaintEventArgs e)
{
}
protected override void Dispose(bool disposing)
{
base.Dispose(disposing);
if (thread != null && thread.ThreadState == ThreadState.Running)
{
thread.Abort();
}
}
}
}<file_sep>/Source/LookAndFeelFramework/ClientApp/Program.cs
using ClientApp.Properties;
using LookAndFeel.Controls;
using LookAndFeel.Factories;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Diagnostics;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace ClientApp
{
static class Program
{
/// <summary>
/// The main entry point for the application.
/// </summary>
static LookAndFeel.Forms.Form form;
static LookAndFeel.Controls.TextBox textbox1, textbox2;
static LookAndFeel.Controls.Label labNotification;
private static LookAndFeel.Controls.CheckBox checkbox;
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
var assembly = AppDomain.CurrentDomain.GetAssemblies().First(a => a.FullName.IndexOf("LookAndFeelLibrary") >= 0);
var type = assembly.GetTypes().First(t => t.Name.Equals(Settings.Default.Factory));
var obj = Activator.CreateInstance(type);
ComponentFactory factory = (ComponentFactory)obj;
ComponentInfo info;
//Form
info = new ComponentInfo(0, 0, 350, 550, "Demo");
form = (LookAndFeel.Forms.Form)factory.create("Form", info);
form.FormClosing += (sender, e) =>
{
if (!Settings.Default.Remember) return;
Settings.Default.Username = textbox1.Text;
Settings.Default.Password = <PASSWORD>;
Settings.Default.Save();
};
//Label username
info = new ComponentInfo(20, 50, 0, 0, "Username");
var lab1 = (LookAndFeel.Controls.IControl)factory.create("Label", info);
//Textbox username
info = new ComponentInfo(20, 75, 150, 25, "");
textbox1 = (LookAndFeel.Controls.TextBox)factory.create("TextBox", info);
//Label password
info = new ComponentInfo(20, 120, 0, 0, "Password");
var lab2 = (LookAndFeel.Controls.IControl)factory.create("Label", info);
//Textbox password
info = new ComponentInfo(20, 145, 150, 25, "");
textbox2 = (LookAndFeel.Controls.TextBox)factory.create("TextBox", info);
//Checkbox remember
info = new ComponentInfo(20, 190, 0, 0, "Remember");
checkbox = (LookAndFeel.Controls.CheckBox)factory.create("CheckBox", info);
checkbox.ClickListener += (sender) =>
{
var cbo = sender as LookAndFeel.Controls.CheckBox;
Settings.Default.Remember = cbo.Checked;
Settings.Default.Save();
};
//Button login
info = new ComponentInfo(20, 240, 100, 0, "Login");
var btn = (LookAndFeel.Controls.Button)factory.create("Button", info);
btn.ClickListener += event_ButtonClick;
//Label notification
info = new ComponentInfo(20, 280, 250, 0, "");
labNotification = (LookAndFeel.Controls.Label)factory.create("Label", info);
//Combobox
info = new ComponentInfo(20, 450, 200, 20, "CboChangeTheme");
var combobox = (LookAndFeel.Controls.ComboBox)factory.create("ComboBox", info);
loadComboboxItems(combobox);
selectDefaultOption(combobox);
combobox.SelectionChangeCommitted += (sender, e) =>
{
var cbo = sender as LookAndFeel.Controls.ComboBox;
var pair = (KeyValuePair<String, String>)cbo.SelectedItem ;
if (!pair.Value.Equals(Settings.Default.Factory))
{
Settings.Default.Factory = pair.Value;
Settings.Default.Save();
form.Close();
Process.Start(Application.ExecutablePath);
}
};
//Group box
info = new ComponentInfo(20, 80, 300, 330, "Login");
var group1 = (LookAndFeel.Controls.GroupBox)factory.create("GroupBox", info);
group1.addControl(lab1);
group1.addControl(lab2);
group1.addControl(labNotification);
group1.addControl(textbox1);
group1.addControl(textbox2);
group1.addControl(btn);
group1.addControl(checkbox);
form.addControl(group1);
form.addControl(combobox);
form.Text = "Demo";
//Load textbox
loadTextboxData();
//form = (LookAndFeel.Forms.Form)form.convert(new LightComponentFactory());
//form = (LookAndFeel.Forms.Form)form.convert(new DarkComponentFactory());
Application.Run(form);
}
private static void loadTextboxData()
{
if (Settings.Default.Remember)
{
textbox1.Text = Settings.Default.Username;
textbox2.Text = Settings.Default.Password;
checkbox.Checked = true;
}
}
static void combobox_SelectedIndexChanged(object sender, EventArgs e)
{
Console.WriteLine("Change");
}
private static void selectDefaultOption(LookAndFeel.Controls.ComboBox combobox)
{
var pivot = Settings.Default.Factory;
foreach (var item in combobox.Items)
{
var pair = (KeyValuePair<string, string>)item ;
if (pair.Value.Equals(pivot))
combobox.SelectedItem = item;
}
}
private static void loadComboboxItems(LookAndFeel.Controls.ComboBox combobox)
{
//extract pairs of key/value from Settings
foreach (var line in Settings.Default.FactoryList)
{
string[] pair = line.Split(new char[]{':'});
KeyValuePair<String, String> p = new KeyValuePair<string,string>(pair[0], pair[1]);
combobox.Items.Add(p);
combobox.DisplayMember = "Key";
}
}
private static void event_ButtonClick(object sender)
{
if (textbox1.Text.Length == 0 ||
textbox2.Text.Length == 0)
labNotification.Text = "Chưa nhập username và password!";
else
labNotification.Text = "Hệ thống đang bảo trì!";
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/DarkComboBox.cs
using LookAndFeel.Components;
using LookAndFeel.Controls;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LookAndFeel.Controls
{
public class DarkComboBox : ComboBox
{
private ComponentInfo info;
public DarkComboBox()
{
}
public DarkComboBox(ComponentInfo info) : base(info)
{
UseCustomBackColor = true;
BackColor = Color.FromArgb(228, 241, 254);
}
public override IComponent clone(ComponentInfo info)
{
var newControl = new DarkComboBox(info);
//set info for newControl
//....
return newControl;
}
protected override void OnClick(EventArgs e)
{
base.OnClick(e);
foreach (var handler in this.clickHandlers)
{
handler(this);
}
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
Pen pen = new Pen(this.Parent.ForeColor, 1);
e.Graphics.DrawRectangle(pen, 0, 0, this.Width-1, this.Height-1);
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/DarkLabel.cs
using LookAndFeel.Components;
using LookAndFeel.Controls;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
namespace LookAndFeel.Controls
{
public class DarkLabel : Label
{
private ComponentInfo info;
public DarkLabel()
{
this.Font = new Font("Segoe UI", 9);
}
public DarkLabel(ComponentInfo info) : base(info)
{
this.Font = new Font("Segoe UI", 9);
}
public override IComponent clone(ComponentInfo info)
{
var newControl = new DarkLabel(info);
//set info for newControl
//....
return newControl;
}
protected override void OnClick(EventArgs e)
{
base.OnClick(e);
foreach (var handler in this.clickHandlers)
{
handler(this);
}
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
Font font = this.Font;
// System.Windows.Forms.TextRenderer.MeasureText(this.Text, font);
e.Graphics.Clear(this.Parent.BackColor);
e.Graphics.DrawString(this.Text, font, new SolidBrush(Color.FromArgb(189, 195, 199)), 0, 0);
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/LightGroupBox.cs
using LookAndFeel.Components;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LookAndFeel.Controls
{
public class LightGroupBox : GroupBox
{
private ComponentInfo info;
public LightGroupBox()
{
}
public LightGroupBox(ComponentInfo info)
: base(info)
{
this.Font = new Font("Segoe UI", 11);
}
public override IComponent clone(ComponentInfo info)
{
var newControl = new LightGroupBox(info);
//set info for newControl
//....
return newControl;
}
protected override void OnClick(EventArgs e)
{
base.OnClick(e);
foreach (var handler in this.clickHandlers)
{
handler(this);
}
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
Pen pen = new Pen(ForeColor, 1f);
int titleHeight = this.Height * 0.2 > 30 ? 30 : (int)(this.Height * 0.2);
SizeF textSize = e.Graphics.MeasureString(this.Text, this.Font);
e.Graphics.Clear(BackColor);
e.Graphics.DrawRectangle(pen, 0, (int)textSize.Height / 2, this.Width - 1 - textSize.Height / 2, this.Height - 1 - textSize.Height / 2);
//e.Graphics.DrawLine(new Pen(ForeColor), 0, 0, this.Width - 1, 1);
//e.Graphics.FillRectangle(new SolidBrush(ForeColor), 0, 0, this.Width - 1, titleHeight);
System.Windows.Forms.TextRenderer.DrawText(e.Graphics, this.Text, this.Font, new Point(2, 0), ForeColor, BackColor);
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Forms/DarkForm.cs
using LookAndFeel.Components;
using LookAndFeel.Factories;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
namespace LookAndFeel.Forms{
/**
*
*/
public class DarkForm : Form {
/**
*
*/
public DarkForm() {
this.backColor = Color.FromArgb(255, 34, 49, 63);
this.foreColor = Color.FromArgb(189, 195, 199);
this.lineColor = Color.FromArgb(89, 171, 227);
}
public DarkForm(ComponentInfo info)
: base(info)
{
this.backColor = Color.FromArgb(255, 34, 49, 63);
this.foreColor = Color.FromArgb(189, 195, 199);
this.lineColor = Color.FromArgb(89, 171, 227);
}
protected override void OnLoad(EventArgs e)
{
base.OnLoad(e);
//cheat window button
//List<System.Windows.Forms.Button> btns = new List<System.Windows.Forms.Button>();
}
/**
* @return
*/
public override IComponent clone(ComponentInfo info)
{
return new DarkForm(info);
}
}
}
<file_sep>/Source/LookAndFeelFramework/ClientApp/FormMain.cs
using LookAndFeel.Controls;
using LookAndFeel.Factories;
using LookAndFeel.Forms;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ClientApp
{
public partial class FormMain : DarkForm
{
public FormMain()
{
InitializeComponent();
}
private void metroButton1_Click(object sender, EventArgs e)
{
}
private void Form1_Load(object sender, EventArgs e)
{
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Factories/ComponentFactory.cs
using LookAndFeel.Components;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LookAndFeel.Factories{
/**
*
*/
public abstract class ComponentFactory {
protected List<IComponent> samples;
/**
* We initalized this.samples in here. So derived class don't have to initalize it anymore.
*/
public ComponentFactory()
{
samples = new List<IComponent>();
}
/**
* Derived class don't have to implement this
* @param type
* @return
*/
public IComponent create(String type, ComponentInfo info) {
foreach (var c in samples)
{
if (c.getType().ToLower().Equals(type.ToLower()))
return c.clone(info);
}
return null;
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Components/ComponentInfo.cs
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LookAndFeelLibrary.Main.Components
{
public class ComponentInfo
{
public int X;
public int Y;
public int Width;
public int Height;
public string Name;
public object Tag;
public ComponentInfo() { }
public ComponentInfo(int x, int y, int width, int height)
{
this.X = x;
this.Y = y;
this.Width = width;
this.Height = height;
}
public ComponentInfo(int p1, int p2, int p3, int p4, string p5)
{
// TODO: Complete member initialization
this.X = p1;
this.Y = p2;
this.Width = p3;
this.Height = p4;
this.Name = p5;
}
public Point Pos {
get {
return new Point(X, Y);
}
}
public SizeF Size {
get {
return new SizeF(Width, Height);
}
}
public ComponentInfo clone()
{
return this.MemberwiseClone() as ComponentInfo;
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/Panel.cs
using LookAndFeel.Components;
using LookAndFeel.Factories;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LookAndFeel.Controls{
/**
*
*/
public class Panel : MetroFramework.Controls.MetroPanel, IControl {
protected List<ClickHandler> clickHandlers;
/**
*
*/
public Panel() {
this.clickHandlers = new List<ClickHandler>();
}
public event ClickHandler ClickListener
{
add
{
this.clickHandlers.Add(value);
}
remove
{
this.clickHandlers.Remove(value);
}
}
/**
* @return
*/
public IComponent clone(ComponentInfo info)
{
// TODO implement here
return null;
}
/**
* @param f
* @return
*/
public IComponent convert(ComponentFactory f) {
// TODO implement here
return null;
}
/**
* @return
*/
public String getType() {
return "Panel";
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/LightLabel.cs
using LookAndFeel.Components;
using LookAndFeel.Controls;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
namespace LookAndFeel.Controls
{
public class LightLabel : Label, IControl
{
public LightLabel()
{
this.Font = new Font("Segoe UI", 9);
}
public LightLabel(ComponentInfo info) : base(info)
{
this.Font = new Font("Segoe UI", 9);
ForeColor = Color.FromArgb(150, 40, 27);
}
public override IComponent clone(ComponentInfo info)
{
var newControl = new LightLabel(info);
//set info for newControl
//....
return newControl;
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
Font font = new System.Drawing.Font("Arial", 9);
e.Graphics.Clear(this.Parent.BackColor);
e.Graphics.DrawString(this.Text, font, new SolidBrush(ForeColor), 0, 0);
}
}
}<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/IContainerControl.cs
using LookAndFeel.Components;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LookAndFeel.Controls{
/**
*
*/
public interface IContainerControl : IControl {
/**
* @param c
*/
void addChildren(IComponent c);
/**
* @return
*/
IComponent[] getChildrens();
/**
* @param c
*/
void removeChildren(IComponent c);
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Components/IComponent.cs
using LookAndFeel.Factories;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LookAndFeel.Components{
/**
*
*/
public interface IComponent {
/**
* @return
*/
IComponent clone(ComponentInfo info);
/**
* @return
*/
String getType();
/**
* @param f
* @return
*/
IComponent convert(ComponentFactory f);
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/TextBox.cs
using LookAndFeel.Components;
using LookAndFeel.Factories;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LookAndFeel.Controls{
/**
*
*/
public abstract class TextBox : MetroFramework.Controls.MetroTextBox, IControl {
private const int WM_PAINT = 15;
private const int OCM_COMMAND = 0x2111;
protected List<ClickHandler> clickHandlers = new List<ClickHandler>();
private ComponentInfo info;
/**
*
*/
public TextBox() {
this.Width = 100;
}
public TextBox(ComponentInfo info)
{
this.info = info.clone();
this.Top = info.Y;
this.Left = info.X;
this.Name = this.Text = info.Name;
this.Width = info.Width != 0 ? info.Width : this.Width;
this.Height = info.Height != 0 ? info.Height : this.Height;
info.Width = info.Width != 0 ? info.Width : this.Width;
info.Height = info.Height != 0 ? info.Height : this.Height;
this.Width = info.Width = this.Width == 0 ? 100 : this.Width;
}
public event ClickHandler ClickListener
{
add
{
this.clickHandlers.Add(value);
}
remove
{
this.clickHandlers.Remove(value);
}
}
/**
* @return
*/
public String getType() {
// TODO implement here
return "TextBox";
}
/**
* @param f
* @return
*/
public IComponent convert(ComponentFactory f) {
// TODO implement here
return null;
}
/**
* @return
*/
public abstract IComponent clone(ComponentInfo info);
protected override void OnTextChanged(EventArgs e)
{
base.OnTextChanged(e);
Invalidate();
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Forms/Form.cs
using LookAndFeel.Components;
using LookAndFeel.Controls;
using LookAndFeel.Factories;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LookAndFeel.Forms{
/**
*
*/
public abstract class Form : MetroFramework.Forms.MetroForm, IComponent {
protected ComponentInfo info;
protected System.Drawing.Color backColor = System.Drawing.Color.White;
protected System.Drawing.Color foreColor = System.Drawing.Color.Black;
protected System.Drawing.Color lineColor = System.Drawing.Color.BlueViolet;
protected Button[] windowButtons = new Button[3];
/**
*
*/
public Form() {
}
public Form(ComponentInfo info)
{
this.info = info.clone();
this.Top = info.Y;
this.Left = info.X;
this.Name = this.Text = info.Name;
this.Width = info.Width >= 0 ? info.Width : this.Width;
this.Height = info.Height >= 0 ? info.Height : this.Height;
info.Width = info.Width >= 0 ? info.Width : this.Width;
info.Height = info.Height >= 0 ? info.Height : this.Height;
AddWindowButtons();
}
private void AddWindowButtons()
{
ComponentInfo info;
Button btn;
info = new ComponentInfo(this.Width - 25, 4, 25, 25, "r");
btn = new WindowButton(info);
btn.Tag = 25;
this.Controls.Add(btn);
btn.Parent = this;
btn.Click += btn_Click;
windowButtons[0] = btn;
info = new ComponentInfo(this.Width - 50, 4, 25, 25, "0");
btn = new WindowButton(info);
btn.Tag = 50;
this.Controls.Add(btn);
btn.Parent = this;
btn.Click += (sender, e) => { this.WindowState = System.Windows.Forms.FormWindowState.Minimized; };
windowButtons[1] = btn;
}
protected override void OnLoad(EventArgs e)
{
base.OnLoad(e);
List<System.Windows.Forms.Control> list = new List<System.Windows.Forms.Control>();
//Extract default window button
foreach (System.Windows.Forms.Control item in this.Controls)
{
if (item.GetType().Name.Equals("MetroFormButton"))
list.Add(item);
}
//remove
foreach (var item in list)
{
this.Controls.Remove(item);
}
this.Font = new System.Drawing.Font("Segoe UI", 8);
Invalidate();
}
protected override void OnResizeEnd(EventArgs e)
{
base.OnResizeEnd(e);
updateWindowButton();
}
protected override void OnResize(EventArgs e)
{
base.OnResize(e);
updateWindowButton();
}
private void updateWindowButton()
{
foreach (var b in windowButtons)
{
if (b != null)
{
b.Left = this.Width - (int)b.Tag;
}
}
}
void btn_Click(object sender, EventArgs e)
{
this.Close();
}
/**
*
*/
protected HashSet<IControl> controls = new HashSet<IControl>();
/**
* @param f
* @return
*/
public IComponent convert(ComponentFactory f)
{
ComponentInfo info = new ComponentInfo();
var newForm = (Form)f.create(this.getType(), info);
foreach (var c in this.controls)
{
newForm.addControl((IControl)c.convert(f));
}
return newForm;
}
public void addControl(IControl component)
{
//((System.Windows.Forms.Control)component).Parent = this;
this.controls.Add(component);
this.Controls.Add((System.Windows.Forms.Control)component);
}
public void removeControl(IControl component)
{
this.controls.Remove(component);
this.Controls.Remove((System.Windows.Forms.Control)component);
}
/**
* @return
*/
public String getType()
{
// TODO implement here
return "Form";
}
public abstract IComponent clone(ComponentInfo info);
public override System.Drawing.Color BackColor
{
get
{
return this.backColor;
}
set
{
this.backColor = value;
}
}
public override System.Drawing.Color ForeColor
{
get
{
return this.foreColor;
}
set
{
this.foreColor = value;
}
}
protected override void OnPaintBackground(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaintBackground(e);
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
e.Graphics.Clear(this.BackColor);
e.Graphics.FillRectangle(new System.Drawing.SolidBrush(this.lineColor),
0, 0, this.Width, 4);
var font = new System.Drawing.Font(this.Font.FontFamily, 12);
e.Graphics.DrawString(this.Text,
font,
new System.Drawing.SolidBrush(this.foreColor),
20, 20);
}
/**
* Nested class WindowButton
**/
private class WindowButton : Button
{
public WindowButton(ComponentInfo info) : base(info)
{
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
var backColor = this.Parent.BackColor;
var foreColor = this.Parent.ForeColor;
if (isHovered)
{
backColor = System.Drawing.Color.FromArgb(
(int)(backColor.R * 0.8f),
(int)(backColor.G * 0.8f),
(int)(backColor.B * 0.8f));
}
e.Graphics.Clear(backColor);
var font = new System.Drawing.Font("Webdings", 9.25f);
System.Windows.Forms.TextRenderer.DrawText(
e.Graphics,
Text,
font,
ClientRectangle,
foreColor,
backColor,
System.Windows.Forms.TextFormatFlags.HorizontalCenter | System.Windows.Forms.TextFormatFlags.VerticalCenter | System.Windows.Forms.TextFormatFlags.EndEllipsis);
}
public override IComponent clone(ComponentInfo info)
{
return new WindowButton(info);
}
}
}
}<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/DarkGroupBox.cs
using LookAndFeel.Components;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LookAndFeel.Controls
{
public class DarkGroupBox : GroupBox
{
private ComponentInfo info;
public DarkGroupBox(){
}
public DarkGroupBox(ComponentInfo info) : base(info)
{
this.Font = new Font("Segoe UI", 11);
}
public override IComponent clone(ComponentInfo info)
{
var newControl = new DarkGroupBox(info);
//set info for newControl
//....
return newControl;
}
protected override void OnClick(EventArgs e)
{
base.OnClick(e);
foreach (var handler in this.clickHandlers)
{
handler(this);
}
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
Pen pen = new Pen(ForeColor, .5f);
int titleHeight = this.Height * 0.2 > 30 ? 30 : (int)(this.Height * 0.2);
e.Graphics.Clear(BackColor);
e.Graphics.DrawRectangle(pen, 0, 0, this.Width-1, this.Height-1);
e.Graphics.FillRectangle(new SolidBrush(ForeColor), 0, 0, this.Width - 1, titleHeight);
SizeF textSize = e.Graphics.MeasureString(this.Text, this.Font);
System.Windows.Forms.TextRenderer.DrawText(e.Graphics, this.Text, this.Font, new Point(2, titleHeight/2 - (int)textSize.Height/2), BackColor);
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/LightTextBox.cs
using LookAndFeel.Components;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LookAndFeel.Controls
{
public class LightTextBox : TextBox
{
private bool focused;
public LightTextBox()
{
}
public LightTextBox(ComponentInfo info)
: base(info)
{
BackColor = Color.FromArgb(255, 246, 237);
FontSize = MetroFramework.MetroTextBoxSize.Medium;
UseCustomBackColor = true;
UseCustomForeColor = true;
Style = MetroFramework.MetroColorStyle.Black;
}
public override IComponent clone(ComponentInfo info)
{
var newControl = new LightTextBox(info);
//set info for newControl
//....
return newControl;
}
protected override void OnEnter(EventArgs e)
{
base.OnEnter(e);
this.focused = true;
Invalidate();
}
protected override void OnLeave(EventArgs e)
{
base.OnLeave(e);
this.focused = false;
Invalidate();
}
protected override void OnClick(EventArgs e)
{
base.OnClick(e);
foreach (var handler in this.clickHandlers)
{
handler(this);
}
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
int lineWidth = 1;
if (focused)
{
lineWidth = 2;
}
Pen pen = new Pen(this.Parent.ForeColor, lineWidth);
Pen clearPen = new Pen(this.Parent.BackColor);
e.Graphics.DrawRectangle(clearPen,
0,
0,
this.Width - 1,
this.Height - 1);
e.Graphics.DrawLine(pen, 0, this.Height - 1, this.Width, this.Height - 1);
e.Graphics.DrawLine(pen, 0, this.Height - 1, 0, this.Height * .7f);
e.Graphics.DrawLine(pen, this.Width - 1, this.Height - 1, this.Width - 1, this.Height * .7f);
}
protected override void OnPaintBackground(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaintBackground(e);
}
protected override void OnGotFocus(EventArgs e)
{
base.OnGotFocus(e);
//this.ForeColor = Color.DarkGray;
}
protected override void OnForeColorChanged(EventArgs e)
{
base.OnForeColorChanged(e);
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/DarkTextBox.cs
using LookAndFeel.Components;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LookAndFeel.Controls
{
public class DarkTextBox : TextBox
{
public DarkTextBox()
{
}
public DarkTextBox(ComponentInfo info) : base(info)
{
BackColor = Color.FromArgb(228, 241, 254);
ForeColor = Color.Red;
FontSize = MetroFramework.MetroTextBoxSize.Medium;
UseCustomBackColor = true;
UseCustomForeColor = true;
Style = MetroFramework.MetroColorStyle.White;
this.ForeColor = Color.FromArgb(34, 49, 63);
}
public override IComponent clone(ComponentInfo info)
{
var newControl = new DarkTextBox(info);
//set info for newControl
//....
return newControl;
}
protected override void OnClick(EventArgs e)
{
base.OnClick(e);
foreach (var handler in this.clickHandlers)
{
handler(this);
}
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
}
protected override void OnPaintBackground(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaintBackground(e);
}
protected override void OnGotFocus(EventArgs e)
{
base.OnGotFocus(e);
//this.ForeColor = Color.DarkGray;
}
protected override void OnForeColorChanged(EventArgs e)
{
base.OnForeColorChanged(e);
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/DarkButton.cs
using LookAndFeel.Components;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
namespace LookAndFeel.Controls
{
/**
*
*/
public class DarkButton : Button
{
/**
*
*/
public DarkButton()
{
this.BackColor = Color.FromArgb(34, 49, 63);
this.ForeColor = Color.FromArgb(189, 195, 199);
this.Font = new Font("Segoe UI", 8);
}
public DarkButton(ComponentInfo info) : base(info)
{
this.BackColor = Color.FromArgb(34, 49, 63);
this.ForeColor = Color.FromArgb(189, 195, 199);
this.Font = new Font("Segoe UI", 8);
}
public override IComponent clone(ComponentInfo info)
{
var newControl = new DarkButton(info);
//set info for newControl
//....
return newControl;
}
protected override void OnClick(EventArgs e)
{
base.OnClick(e);
foreach (var handler in this.clickHandlers)
{
handler(this);
}
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
//Draw border
//Brush b = new SolidBrush(this.BackColor);
//e.Graphics.DrawRectangle(new Pen(Color.FromArgb(51, 110, 123), 2), 0, 0, this.Width, this.Height);
try
{
base.OnPaintBackground(e);
Color backColor = BackColor;
Color foreColor = ForeColor;
Color borderColor = Color.FromArgb(
Math.Min((int)(foreColor.R * .7f), 255),
Math.Min((int)(foreColor.G * .7f), 255),
Math.Min((int)(foreColor.B * .7f), 255));
if (isHovered && !isPressed && Enabled)
{
backColor = Color.FromArgb(
(int)(BackColor.R * 0.5f),
(int)(BackColor.G * 0.5f),
(int)(BackColor.B * 0.5f)
);
}
else if (isHovered && isPressed && Enabled)
{
//backColor = Color.FromArgb(44, 62, 80);
//foreColor = Color.FromArgb(239, 245, 249);
}
else if (Focused)
{
foreColor = Color.White;
}
if (backColor.A == 255 && BackgroundImage == null)
{
e.Graphics.Clear(backColor);
}
e.Graphics.DrawRectangle(new Pen(borderColor), 0, 0, this.Width - 1, this.Height - 1);
System.Windows.Forms.TextRenderer.DrawText(e.Graphics,
this.Text, this.Font,
new Point(this.Width, this.Height/2),
foreColor,
Color.Transparent,
System.Windows.Forms.TextFormatFlags.HorizontalCenter | System.Windows.Forms.TextFormatFlags.VerticalCenter);
}
catch
{
Invalidate();
}
}
protected override void OnPaintBackground(System.Windows.Forms.PaintEventArgs e)
{
}
}
}<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/GroupBox.cs
using LookAndFeel.Components;
using LookAndFeel.Factories;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LookAndFeel.Controls{
/**
*
*/
public abstract class GroupBox : System.Windows.Forms.GroupBox , IControl {
protected HashSet<IControl> controls = new HashSet<IControl>();
protected List<ClickHandler> clickHandlers = new List<ClickHandler>();
protected ComponentInfo info;
/**
*
*/
public GroupBox() {
}
public GroupBox(ComponentInfo info)
{
this.info = info.clone();
this.Top = info.Y;
this.Left = info.X;
this.Name = this.Text = info.Name;
this.Width = info.Width != 0 ? info.Width : this.Width;
this.Height = info.Height != 0 ? info.Height : this.Height;
info.Width = info.Width != 0 ? info.Width : this.Width;
info.Height = info.Height != 0 ? info.Height : this.Height;
this.ParentChanged += (sender, e) => {
var parent = ((GroupBox)sender).Parent;
if (parent != null)
{
BackColor = parent.BackColor;
ForeColor = parent.ForeColor;
}
};
}
public event ClickHandler ClickListener
{
add
{
this.clickHandlers.Add(value);
}
remove
{
this.clickHandlers.Remove(value);
}
}
/**
* @param f
* @return
*/
public IComponent convert(ComponentFactory f) {
// TODO implement here
return null;
}
/**
* @return
*/
public abstract IComponent clone(ComponentInfo info);
/**
* @return
*/
public String getType() {
return "GroupBox";
}
public void addControl(IControl component)
{
this.controls.Add(component);
this.Controls.Add((System.Windows.Forms.Control)component);
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/Button.cs
using LookAndFeel.Components;
using LookAndFeel.Factories;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LookAndFeel.Controls{
/**
*
*/
public abstract class Button : MetroFramework.Controls.MetroButton, IControl {
protected bool isHovered;
protected bool isPressed;
protected List<ClickHandler> clickHandlers = new List<ClickHandler>();
protected ComponentInfo info;
/**
*
*/
public Button() {
info = new ComponentInfo();
}
public Button(ComponentInfo info)
{
// TODO: Complete member initialization
this.info = info.clone();
this.Top = info.Y;
this.Left = info.X;
this.Name = this.Text = info.Name;
this.Width = info.Width != 0 ? info.Width : this.Width;
this.Height = info.Height != 0 ? info.Height : this.Height;
info.Width = info.Width != 0 ? info.Width : this.Width;
info.Height = info.Height != 0 ? info.Height : this.Height;
}
/**
* @return
*/
public String getType() {
return "Button";
}
/**
* @return
*/
public abstract IComponent clone(ComponentInfo info);
/**
* @param f
* @return
*/
public IComponent convert(ComponentFactory f) {
return f.create(this.getType(), this.info);
}
public event ClickHandler ClickListener
{
add{
this.clickHandlers.Add(value);
}
remove {
this.clickHandlers.Remove(value);
}
}
protected override void OnMouseDown(System.Windows.Forms.MouseEventArgs e)
{
base.OnMouseDown(e);
isPressed = true;
}
protected override void OnMouseUp(System.Windows.Forms.MouseEventArgs e)
{
base.OnMouseUp(e);
isPressed = false;
Invalidate();
}
protected override void OnMouseEnter(EventArgs e)
{
base.OnMouseEnter(e);
isHovered = true;
}
protected override void OnMouseLeave(EventArgs e)
{
base.OnMouseLeave(e);
isHovered = false;
}
public override string Text
{
get
{
return base.Text;
}
set
{
this.Name = value;
base.Text = value;
}
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Factories/DarkComponentFactory.cs
using LookAndFeel.Controls;
using LookAndFeel.Forms;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LookAndFeel.Factories{
/**
*
*/
public class DarkComponentFactory : ComponentFactory {
/**
* You need add component samples in here
*/
public DarkComponentFactory() {
this.samples.Add(new DarkButton());
this.samples.Add(new DarkForm());
this.samples.Add(new DarkLabel());
this.samples.Add(new DarkCheckBox());
this.samples.Add(new DarkComboBox());
this.samples.Add(new DarkTextBox());
this.samples.Add(new DarkGroupBox());
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/LightComboBox.cs
using LookAndFeel.Components;
using LookAndFeel.Controls;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LookAndFeel.Controls
{
public class LightComboBox : ComboBox
{
private ComponentInfo info;
public LightComboBox()
{
UseCustomBackColor = true;
BackColor = Color.FromArgb(241, 169, 160);
}
public LightComboBox(ComponentInfo info)
: base(info)
{
UseCustomBackColor = true;
BackColor = Color.FromArgb(255, 246, 237);
}
public override IComponent clone(ComponentInfo info)
{
var newControl = new LightComboBox(info);
//set info for newControl
//....
return newControl;
}
protected override void OnClick(EventArgs e)
{
base.OnClick(e);
foreach (var handler in this.clickHandlers)
{
handler(this);
}
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
Pen pen = new Pen(this.Parent.ForeColor, 1);
e.Graphics.DrawRectangle(pen, 0, 0, this.Width - 1, this.Height - 1);
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Forms/LightForm.cs
using LookAndFeel.Components;
using LookAndFeel.Factories;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
namespace LookAndFeel.Forms{
/**
*
*/
public class LightForm : Form {
/**
*
*/
public LightForm() {
this.backColor = Color.FromArgb(34, 49, 63);
this.foreColor = Color.FromArgb(189, 195, 199);
this.lineColor = Color.FromArgb(89, 171, 227);
}
public LightForm(ComponentInfo info) : base(info)
{
this.backColor = Color.White;
this.foreColor = Color.FromArgb(150, 40, 27);
this.lineColor = Color.FromArgb(217, 30, 24);
}
/**
* @return
*/
public override IComponent clone(ComponentInfo info)
{
return new LightForm(info);
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Factories/LightComponentFactory.cs
using LookAndFeel.Controls;
using LookAndFeel.Forms;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LookAndFeel.Factories{
/**
*
*/
public class LightComponentFactory : ComponentFactory {
/**
* You need add component samples in here
*/
public LightComponentFactory() {
this.samples.Add(new LightButton());
this.samples.Add(new LightForm());
this.samples.Add(new LightLabel());
this.samples.Add(new LightCheckBox());
this.samples.Add(new LightComboBox());
this.samples.Add(new LightTextBox());
this.samples.Add(new LightGroupBox());
}
}
}<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/LightCheckBox.cs
using LookAndFeel.Components;
using LookAndFeel.Controls;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LookAndFeel.Controls
{
public class LightCheckBox : CheckBox
{
private ComponentInfo info;
public LightCheckBox()
{
this.Font = new Font("Segoe UI", 8);
}
public LightCheckBox(ComponentInfo info)
: base(info)
{
this.Font = new Font("Segoe UI", 8);
}
public override IComponent clone(ComponentInfo info)
{
var newControl = new LightCheckBox(info);
//set info for newControl
//....
return newControl;
}
protected override void OnClick(EventArgs e)
{
base.OnClick(e);
foreach (var handler in this.clickHandlers)
{
handler(this);
}
}
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
Color foreColor = this.Parent.ForeColor;
Color backColor;
if (isHovered)
foreColor = Color.FromArgb(
Math.Min((int)(foreColor.R * 1.3f), 255),
Math.Min((int)(foreColor.G * 1.3f), 255),
Math.Min((int)(foreColor.B * 1.3f), 255));
backColor = Color.FromArgb(
(int)(foreColor.R * .7f),
(int)(foreColor.G * .7f),
(int)(foreColor.B * .7f));
SizeF textSize = e.Graphics.MeasureString(this.Text, this.Font);
e.Graphics.Clear(this.Parent.BackColor);
System.Windows.Forms.TextRenderer.DrawText(e.Graphics, this.Text, this.Font, new Point(14, (int)(this.Height / 2 - textSize.Height / 2)), foreColor);
e.Graphics.DrawRectangle(new Pen(backColor), 0, this.Height / 4 - 1, this.Height / 2, this.Height / 2);
if (this.Checked)
{
e.Graphics.FillRectangle(new SolidBrush(backColor),
(int)(this.Height / 4 * 0.4),
(int)(this.Height / 4 * 1.4 - 1),
(int)(this.Height / 2 * 0.8),
(int)(this.Height / 2 * 0.8));
}
}
}
}
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/IControl.cs
using LookAndFeel.Components;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace LookAndFeel.Controls{
/**
*
*/
public delegate void ClickHandler(object sender);
public interface IControl : IComponent {
event ClickHandler ClickListener;
}
}
<file_sep>/README.md
# LookAndFeel
Thiết kế và phát triển dự án LookAndFeel
<file_sep>/Source/LookAndFeelFramework/LookAndFeelLibrary/Main/Controls/ComboBox.cs
using LookAndFeel.Components;
using LookAndFeel.Controls;
using LookAndFeel.Factories;
using LookAndFeelLibrary.Main.Components;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LookAndFeel.Controls
{
public abstract class ComboBox : MetroFramework.Controls.MetroComboBox, IControl
{
protected List<ClickHandler> clickHandlers = new List<ClickHandler>();
protected ComponentInfo info;
public ComboBox()
{
}
public ComboBox(ComponentInfo info)
{
this.info = info.clone();
this.Top = info.Y;
this.Left = info.X;
this.Name = this.Text = info.Name;
this.Width = info.Width != 0 ? info.Width : this.Width;
this.Height = info.Height != 0 ? info.Height : this.Height;
info.Width = info.Width != 0 ? info.Width : this.Width;
info.Height = info.Height != 0 ? info.Height : this.Height;
}
public event ClickHandler ClickListener
{
add
{
this.clickHandlers.Add(value);
}
remove
{
this.clickHandlers.Remove(value);
}
}
/**
* @return
*/
public String getType() {
return "ComboBox";
}
/**
* @return
*/
public abstract IComponent clone(ComponentInfo info);
/**
* @param f
* @return
*/
public IComponent convert(ComponentFactory f) {
var info = new ComponentInfo();
return f.create(this.getType(), info);
}
}
}
| b1d49ac099117adf004c011fc97e8345931a776e | [
"Markdown",
"C#"
] | 29 | C# | MTKGroup/LookAndFeel | f9c13940f144ea4be5e45489006c4d3cc53e6f88 | a9aa56e5b1ec97daa3aafba882400e7535b55777 | |
refs/heads/main | <repo_name>ChristianDarlington/React-Practice-First-Day<file_sep>/src/components/Menu.js
import React from 'react'
import MenuItems from './MenuItem'
function Menu() {
return (
<ul>
<MenuItems item="Chicken Fingers" />
<MenuItems item="Fried Chicken" />
<MenuItems item="Noodles" />
<MenuItems item="Soup" />
</ul>
)
}
export default Menu <file_sep>/src/components/MainImage.js
import React from 'react';
import heroImage from '../Image/Meatball.jpeg'
function MainImage(){
return(
<div className="image-container">
<img className="main-image" src={heroImage} />
<img src={heroImage} />
</div>
)
}
export default MainImage
<file_sep>/src/components/FoodItem.js
import React from 'react';
function FoodItem (props){
return(
<div>
<h5>{props.item.foodName}</h5>
<p>{props.item.foodName}</p>
<p><strong>{props.item.price}</strong></p>
</div>
)
}
export default FoodItem<file_sep>/src/components/Header.js
import React from 'react'
function Header() {
return(
<>
<h4 className="header">Sanchise Meatballs</h4>
</>
)
}
export default Header <file_sep>/src/components/FoodItems.js
import React from 'react';
import FoodItem from './FoodItem';
const food = [
{
id:1,
foodName: "Italian Combo",
price:"$12.99",
ingredients:"deli meat, lettuce, tomato, bread, provolone"
},
{
id:2,
foodName:"Pastrami Sandwhich",
price:"$13.99",
ingredients:"pastrami, rye bread"
},
{
id:3,
foodName:"Peanut Butter and Jelly",
price:"$4.99",
ingredients:"Peanutbutter, Grape Jelly, Bread"
}
]
function FoodItems () {
return(
<div>
{food.map(sandwich => <FoodItem key={sandwich.id} item={sandwich} />)}
</div>
)
}
export default FoodItems | a917779e00ffd24ce6620474d2fb8480fb1e1db4 | [
"JavaScript"
] | 5 | JavaScript | ChristianDarlington/React-Practice-First-Day | e7384239ccc33781de801fae552db8277e1bf61b | c0988afdce67c4a9cd2ad4ec1142b8fb5e59e716 | |
refs/heads/master | <file_sep>
namespace TSP
{
interface Solver
{
Problem solve(Problem cityData);
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace TSP
{
class Problem
{
// Default values
public const int DEFAULT_SEED = 1;
public const int DEFAULT_PROBLEM_SIZE = 20;
public const int DEFAULT_TIME_LIMIT = 60; // in seconds
private const double FRACTION_OF_PATHS_TO_REMOVE = 0.20;
// Class members
public int seed;
public int size;
public int timeLimit;
public HardMode.Modes mode;
private Random rnd;
public City[] cities;
public TSPSolution bssf;
public TimeSpan timeElasped;
public int solutions;
// Constructors
public Problem()
{
initialize(DEFAULT_SEED, DEFAULT_PROBLEM_SIZE, DEFAULT_TIME_LIMIT, HardMode.Modes.Hard);
}
public Problem(int seed, int size, int timeInSeconds, HardMode.Modes mode)
{
initialize(seed, size, timeInSeconds, mode);
}
private void initialize(int seed, int problemSize, int timeInSeconds, HardMode.Modes mode)
{
this.seed = seed;
this.rnd = new Random(seed);
// CRITICAL - TO MAKE THE POINTS LOOK LIKE THE
// POINTS IN THE OLD VERSION FOR THE SAME SEEDS
// DO NOT REMOVE THIS FOR LOOP
for (int i = 0; i < 50; i++)
rnd.NextDouble();
this.size = problemSize;
this.mode = mode;
this.timeLimit = timeInSeconds * 1000; // timer wants timeLimit in milliseconds
this.resetData();
}
// Reset the problem instance
private void resetData()
{
cities = new City[size];
bssf = null;
if (mode == HardMode.Modes.Easy)
{
for (int i = 0; i < size; i++)
cities[i] = new City(rnd.NextDouble(), rnd.NextDouble());
}
else // Medium and hard
{
for (int i = 0; i < size; i++)
cities[i] = new City(rnd.NextDouble(), rnd.NextDouble(), rnd.NextDouble() * City.MAX_ELEVATION);
}
HardMode mm = new HardMode(this.mode, this.rnd, cities);
if (mode == HardMode.Modes.Hard)
{
int edgesToRemove = (int)(size * FRACTION_OF_PATHS_TO_REMOVE);
mm.removePaths(edgesToRemove);
}
City.setModeManager(mm);
}
// Return the cost of the best solution so far.
public double costOfBssf()
{
if (bssf != null)
{
// go through each edge in the route and add up the cost.
int x;
List<City> route = bssf.route;
City here;
double cost = 0D;
for (x = 0; x < route.Count - 1; x++)
{
here = route[x];
cost += here.costToGetTo(route[x + 1]);
}
// go from the last city to the first.
here = route[route.Count - 1];
cost += here.costToGetTo(route[0]);
return cost;
}
else
return -1D;
}
}
}
<file_sep>using System;
namespace TSP
{
class Test
{
public static void run()
{
bBHardLastCityBlocked();
greedyHard();
bbHardBetterThanGreedy();
}
public static void greedyEasy()
{
Problem cityData = new Problem(1, 5, 60, HardMode.Modes.Easy);
cityData = GreedySolver.solve(cityData);
if (cityData.bssf.costOfRoute != 2060)
throw new SystemException("Incorrect cost");
}
public static void greedyEasy2()
{
Problem cityData = new Problem(1, 20, 60, HardMode.Modes.Hard);
cityData = GreedySolver.solve(cityData);
if (cityData.bssf.costOfRoute != 4484)
throw new SystemException("Incorrect cost");
}
//This case should return the greedy value bc that is best
public static void bBEasy()
{
Problem cityData = new Problem(1, 5, 60, HardMode.Modes.Easy);
BranchAndBoundSolver solver = new BranchAndBoundSolver(cityData);
cityData = solver.solve();
if (cityData.bssf.costOfRoute != 2060)
throw new SystemException("Incorrect cost");
}
// There exists a better path than the greedy one
public static void bBNormal()
{
Problem cityData = new Problem(268, 16, 60, HardMode.Modes.Normal);
BranchAndBoundSolver solver = new BranchAndBoundSolver(cityData);
cityData = solver.solve();
if (cityData.bssf.costOfRoute != 4069)
throw new SystemException("Incorrect cost");
}
// The last city is blocked
// Ends up using the greedy value
public static void bBHardLastCityBlocked()
{
Problem cityData = new Problem(1, 5, 60, HardMode.Modes.Hard);
BranchAndBoundSolver solver = new BranchAndBoundSolver(cityData);
cityData = solver.solve();
if (cityData.bssf.costOfRoute != 2123)
throw new SystemException("Incorrect cost");
}
public static void greedyHard()
{
Problem cityData = new Problem(1, 20, 60, HardMode.Modes.Hard);
cityData = GreedySolver.solve(cityData);
if (cityData.bssf.costOfRoute != 4928)
throw new SystemException("Incorrect cost");
}
public static void bbHardBetterThanGreedy()
{
Problem cityData = new Problem(1, 8, 60, HardMode.Modes.Hard);
BranchAndBoundSolver solver = new BranchAndBoundSolver(cityData);
cityData = solver.solve();
if (cityData.bssf.costOfRoute != 3015)
throw new SystemException("Incorrect cost");
}
}
}
<file_sep>using System.Diagnostics;
namespace TSP
{
/////////////////////////////////////////////////////////////////////////////////////////////
// Project 6
////////////////////////////////////////////////////////////////////////////////////////////
/*
* Implement the greedy solver in the solve() method of this class.
*/
static class GreedySolver
{
// finds the greedy tour starting from each city and keeps the best (valid) one
// For an example of what to return, see DefaultSolver.solve() method.
public static Problem solve(Problem cityData)
{
Stopwatch timer = new Stopwatch();
timer.Start();
State state = new State(cityData.size);
SolverHelper.initializeState(state, cityData);
state.currCityIndex = 0;
recur(state);
timer.Stop();
// Since cityData is static, it is pointing to the cityData object
// that was passed in. Setting it's BSSF here will set the BSSF
// of the cityData object in the Form1 class.
cityData.bssf = new TSPSolution(cityData.cities, state.cityOrder, state.lowerBound);
cityData.timeElasped = timer.Elapsed;
cityData.solutions = 1;
return cityData;
}
// Returns the index of the last city to be visited in the cycle
private static void recur(State state)
{
//state.printState();
int currCityIndex = state.currCityIndex;
// All cities have been visited; finish and return
if(state.getCitiesVisited() == state.size - 1)
{
// The current path cannot be completed bc there is no path from the last city to the first
if (state.matrix[currCityIndex, state.cityOfOriginIndex] == double.PositiveInfinity)
{
state.lowerBound = double.PositiveInfinity;
}
else
{
state.addCity(currCityIndex);
state.lowerBound += state.matrix[currCityIndex, state.cityOfOriginIndex];
return;
}
}
state.addCity(currCityIndex);
// find the smallest distance to any other city
double closestCityDistance = double.PositiveInfinity;
int closestCityIndex = -1;
for (int j = 1; j < state.matrix.GetLength(1); j++)
// For a different starting city, the following code
// would have to be added:
//for (int j = 0; j < state.matrix.GetLength(1); j++)
//if (j == state.startCityIndex)
// continue;
if (state.matrix[currCityIndex, j] < closestCityDistance)
{
closestCityIndex = j;
closestCityDistance = state.matrix[currCityIndex, j];
}
// add [i,j]
state.lowerBound += closestCityDistance;
// Blank out appropriate spaces
SolverHelper.blankOut(state, closestCityIndex);
// reduce
SolverHelper.reduce(state);
state.currCityIndex = closestCityIndex;
recur(state);
}
}
}
<file_sep>using System;
using Newtonsoft.Json;
namespace TSP
{
static class SolverHelper
{
public const double SPACE_PLACEHOLDER = double.PositiveInfinity;
// Example: https://gist.github.com/TangChr/4711193c87f40c9fb18d
public static T clone<T>(T source)
{
var json = JsonConvert.SerializeObject(source);
return JsonConvert.DeserializeObject<T>(json);
}
// Populate the state matrix with distances between cities
public static void initializeState(State state, Problem cityData)
{
for (int i = 0; i < cityData.size; i++)
{
City city = cityData.cities[i];
for (int j = 0; j < cityData.size; j++)
{
if (i == j)
state.matrix[i, j] = SPACE_PLACEHOLDER;
else
state.matrix[i, j] = city.costToGetTo(cityData.cities[j]);
}
}
reduce(state);
}
// Blank out row and column
public static void blankOut(State state, int otherCityIndex)
{
// row i
for (int j = 0; j < state.matrix.GetLength(1); j++)
state.matrix[state.currCityIndex, j] = SolverHelper.SPACE_PLACEHOLDER;
// column j
for (int i = 0; i < state.matrix.GetLength(0); i++)
state.matrix[i, otherCityIndex] = SolverHelper.SPACE_PLACEHOLDER;
// [j,i]
state.matrix[otherCityIndex, state.currCityIndex] = SolverHelper.SPACE_PLACEHOLDER;
}
// Makes sure that there is a 0 in every row and column
public static void reduce(State state)
{
// Reduce the rows
for (int i = 0; i < state.matrix.GetLength(0); i++)
{
// Find the smallest value in the row
double minInRow = double.PositiveInfinity;
for (int j = 0; j < state.matrix.GetLength(1); j++)
minInRow = Math.Min(minInRow, state.matrix[i, j]);
// If the smallest value in the row is 0,
// then there already is a 0 in the row
// We don't need to add a 0 into the row
//
// If the smallest value in the row is infinity,
// then we have a row of infinities
// Nothing happens
if (minInRow != 0 && minInRow != SPACE_PLACEHOLDER)
{
// Add the smallest value in the row to the lower bound
state.lowerBound += minInRow;
// Subtract that value from all the values in the row
// The [i,j] that contained the smallest value will be
// set to 0, bc minInRow - minInRow = 0;
// If the value at [i,j] is the placeholder, nothing happens
for (int j = 0; j < state.matrix.GetLength(1); j++)
if (state.matrix[i, j] != SPACE_PLACEHOLDER)
state.matrix[i, j] -= minInRow;
}
}
// Reduce the columns
for (int j = 0; j < state.matrix.GetLength(1); j++)
{
// Find the smallest value in the column
double minInColumn = double.PositiveInfinity;
for (int i = 0; i < state.matrix.GetLength(0); i++)
minInColumn = Math.Min(minInColumn, state.matrix[i, j]);
// Same as above, except switching rows for columns
if (minInColumn != 0 && minInColumn != SPACE_PLACEHOLDER)
{
// Add the smallest value in the column to the lower bound
state.lowerBound += minInColumn;
// Subtract that value from all the values in the column
for (int i = 0; i < state.matrix.GetLength(0); i++)
if (state.matrix[i, j] != SPACE_PLACEHOLDER)
state.matrix[i, j] -= minInColumn;
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Diagnostics;
namespace TSP
{
class DefaultSolver : Solver
{
// This is the entry point for the default solver
// which just finds a valid random tour
// <returns>The random valid tour</returns>
public Problem solve(Problem cityData)
{
int i, swap, temp = 0;
City[] cities = cityData.cities;
string[] results = new string[3];
int[] perm = new int[cities.Length];
List<City> route = new List<City>();
Random rnd = new Random();
Stopwatch timer = new Stopwatch();
timer.Start();
do
{
for (i = 0; i < perm.Length; i++) // create a random permutation template
perm[i] = i;
for (i = 0; i < perm.Length; i++)
{
swap = i;
while (swap == i)
swap = rnd.Next(0, cities.Length);
temp = perm[i];
perm[i] = perm[swap];
perm[swap] = temp;
}
route.Clear();
for (i = 0; i < cities.Length; i++) // Now build the route using the random permutation
{
route.Add(cities[perm[i]]);
}
cityData.bssf = new TSPSolution(route);
} while (cityData.costOfBssf() == double.PositiveInfinity); // until a valid route is found
timer.Stop();
cityData.bssf.costOfRoute = cityData.costOfBssf();
cityData.timeElasped = timer.Elapsed;
cityData.solutions = 1;
return cityData;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace TSP
{
class State
{
public int size;
public int cityOfOriginIndex;
public int currCityIndex;
public double[,] matrix;
public double lowerBound;
public List<int> cityOrder;
public State(int size)
{
this.size = size;
this.cityOfOriginIndex = 0;
this.currCityIndex = 0;
this.matrix = new double[size, size];
this.lowerBound = 0;
this.cityOrder = new List<int>(size+1);
}
public void addCity(int cityIndex)
{
this.cityOrder.Add(cityIndex);
}
public int getCitiesVisited()
{
return this.cityOrder.Count;
}
public void printState()
{
for (int i = 0; i < matrix.GetLength(0); i++)
{
for (int j = 0; j < matrix.GetLength(1); j++)
{
if (matrix[i, j] == 0)
Console.Write("00000");
else if (matrix[i, j] == double.PositiveInfinity)
Console.Write("infin");
else if (matrix[i, j] < 10)
{
Console.Write("0000");
Console.Write(matrix[i, j]);
}
else if (matrix[i, j] < 100)
{
Console.Write("000");
Console.Write(matrix[i, j]);
}
else if (matrix[i, j] < 1000)
{
Console.Write("00");
Console.Write(matrix[i, j]);
}
else if (matrix[i, j] < 10000)
{
Console.Write("0");
Console.Write(matrix[i, j]);
}
else
Console.Write(matrix[i, j]);
Console.Write(',');
}
Console.Write("\n");
}
Console.Write("\n\n");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace TSP
{
class TSPSolution
{
// we use the representation [cityB,cityA,cityC]
// to mean that cityB is the first city in the solution, cityA is the second, cityC is the third
// and the edge from cityC to cityB is the final edge in the path.
// You are, of course, free to use a different representation if it would be more convenient or efficient
// for your data structure(s) and search algorithm.
public List<City> route;
public double costOfRoute;
public TSPSolution(City[] cities, List<int> cityOrder, double costOfRoute)
{
// From the list of city indexes, create the list of cities
route = new List<City>(cityOrder.Count);
foreach (int i in cityOrder)
{
City temp = SolverHelper.clone(cities[i]);
route.Add(temp);
}
this.costOfRoute = costOfRoute;
}
// <param name="iroute">a (hopefully) valid tour</param>
public TSPSolution(List<City> iroute)
{
route = new List<City>(iroute);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Diagnostics;
namespace TSP
{
/////////////////////////////////////////////////////////////////////////////////////////////
// Project 5
////////////////////////////////////////////////////////////////////////////////////////////
/*
* Implement your branch and bound solver in the solve() method of this class.
*/
class BranchAndBoundSolver
{
Problem cityData;
Queue<State> q;
public BranchAndBoundSolver(Problem cityData)
{
this.cityData = cityData;
q = new Queue<State>();
}
// finds the best tour possible using the branch and bound method of attack
// For an example of what to return, see DefaultSolver.solve() method.
public Problem solve()
{
Stopwatch timer = new Stopwatch();
timer.Start();
State beginningState = new State(cityData.size);
SolverHelper.initializeState(beginningState, cityData);
this.q.Enqueue(beginningState);
Problem determineBSSFProblem = SolverHelper.clone(cityData);
this.cityData.bssf = GreedySolver.solve(determineBSSFProblem).bssf;
while (q.Count > 0)
{
recur(q.Dequeue());
//Clear out any states that have a BSSF less than
//that of the current
}
timer.Stop();
cityData.timeElasped = timer.Elapsed;
return cityData;
}
private void recur(State state)
{
// This is a leaf
// All cities have been visited; finish and return
if (state.getCitiesVisited() == cityData.size - 1)
{
// The path to the last city is blocked
if (state.matrix[state.currCityIndex, state.cityOfOriginIndex]
== SolverHelper.SPACE_PLACEHOLDER)
return;
state.addCity(state.currCityIndex);
// Since we've hit a leaf node, we've found another possible solution
cityData.solutions++;
// Add the last hop to the lower bound to get the cost of the complete cycle
state.lowerBound += state.matrix[state.currCityIndex, state.cityOfOriginIndex];
// Check to see if this route is smaller than the current BSSF
// If so, replace the current BSSF
if (state.lowerBound < cityData.bssf.costOfRoute)
cityData.bssf = new TSPSolution(cityData.cities, state.cityOrder, state.lowerBound);
return;
}
state.addCity(state.currCityIndex);
for (int j = 1; j < state.matrix.GetLength(1); j++)
{
//Console.WriteLine(state.currCityIndex + " " + j);
//state.printState();
double currDistVal = state.matrix[state.currCityIndex, j];
if (currDistVal != SolverHelper.SPACE_PLACEHOLDER &&
state.lowerBound + currDistVal < cityData.bssf.costOfRoute)
{
State childState = SolverHelper.clone(state);
// add [i,j]
childState.lowerBound += currDistVal;
// Blank out the appropriate values with the placeholder
SolverHelper.blankOut(childState, j);
//childState.printState();
// reduce
SolverHelper.reduce(childState);
childState.currCityIndex = j;
if(childState.lowerBound < cityData.bssf.costOfRoute)
q.Enqueue(childState);
}
}
}
}
}
| b709a3f4b04d24f3ab796449f98b2aa2911f4d8d | [
"C#"
] | 9 | C# | jtcotton63/CS312-Project05-REV00 | 2367bab3180875c14cf9140c950e4f136827121c | 1864441fc67d2c55926843b14b46965e592bb99c | |
refs/heads/main | <file_sep>const ENV = process.argv;
const APP_PORT = process.env.APP_PORT || 3005;
const config = {
APP_PORT,
ENV,
};
module.exports = config;<file_sep># lohika-training-nodejs
Nodejs training course
### Run project
- `npm start`
## 1. Homework #1:
- Create the app.js file with the server example (may be copied from the slides) which accepts requests and responds with “Hellow world!” message to all requests.
> - server on port APP_PORT which responds with “Hello world!” message to all requests.
- Take APP_PORT (for server) from env variables, ENV from cli args and populate config object which will be imported in the app.js from config.js.
> - APP_PORT env variable
> - ENV cli arg
> - config.js with config object which contains APP_PORT, ENV
- When server is up – log a message: `Server is listening on port <PORT>. Env is <ENV>.` Use the logger created in a separate file which will use console methods for now
> - log a message `Server is listening on port <PORT>. Env is <ENV>.` Using the imported logger (from separate file)
<file_sep>const http = require('http');
const config = require('./config');
const logger = require('./logger');
const {
APP_PORT: PORT,
ENV,
} = config;
http.createServer((req, res) => {
console.log('New incoming request');
res.writeHeader(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'Hello world!' }));
}).listen(PORT, () => logger.log(`Listening on port ${PORT}... Env is ${ENV}`));
| 5152ad2e2d4be01d3753ca5dde3579f38d2a3cd6 | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | yarikRed/lohika-training-nodejs | 03adc12525d40c3205dc668781f03d8f3efed30d | aa6c6d54617bceca02e081edec11314ec42d7f4f | |
refs/heads/master | <file_sep>//
// Calculator.swift
// ClassDemo
//
// Created by <NAME> on 17/04/21.
//
import Foundation
let calculator = Calculations(num1: 10, num2: 10)
//calculator.division()
//calculator.multiplication()
//calculator.subtraction()
let r = calculator.addition()
<file_sep>
let newEnemy = Enemy()
var one = newEnemy.attackStrength
var two = newEnemy.health
var three = newEnemy.move()
var five = newEnemy.attack()
let dragon = Dragon()
var tr = dragon.attack()
let calculator = Calculations(num1: 5, num2: 7)
print(calculator.division())
print(calculator.multiplication())
print(calculator.subtraction())
print(calculator.addition())
let calculator1 = calculator //here we just made a copy of calculator
print(calculator1.addition())
print(calculator1.value(value: "values"))
let calculator3 = Calculations(num1: 6, num2: 0)
print(calculator3.value(value: "values"))
<file_sep>
class Enemy {
var health = 100
var attackStrength = 10
func move(){
print("Move frswswmwrd")
}
func attack(){
print("Attack lvel is \(attackStrength)")
}
}
<file_sep>//
// Calculations.swift
// ClassDemo
//
// Created by <NAME> on 17/04/21.
//
import Foundation
class Calculations {
var num1 : Int
var num2 : Int
init(num1 : Int, num2 : Int) {
self.num1 = num1
self.num2 = num2
}
func addition() -> Int{
return num1 + num2
}
func subtraction() -> Int{
return num1 - num2
}
func multiplication() -> Int{
return num1 * num2
}
func division() -> Int{
return num1 / num2
}
func value (value : String) -> String{
return ("\(value) are \(num1) and \(num2)")
}
}
<file_sep>//
// Dragon.swift
// ClassDemo
//
// Created by <NAME> on 17/04/21.
//
import Foundation
class Dragon : Enemy {
override func move() {
super.move()
}
override func attack() {
super.attack()
print("attack dragons \(attackStrength)")
}
}
| 3a2ccab6d25b35032b9edeb4f52e365a39d07feb | [
"Swift"
] | 5 | Swift | ALISHA-JOSHI/class-demo-commandline | 88bd9eabc681416aaeab8b67477ea61555902af6 | 387541dea3af803d939376e6ca02382d5700ff65 | |
refs/heads/master | <file_sep><?php
namespace Marmelab\Microrest;
use Raml\Parser;
use Silex\Application;
use Silex\ServiceProviderInterface;
class MicrorestServiceProvider implements ServiceProviderInterface
{
public function register(Application $app)
{
$app['microrest.initializer'] = $app->protect(function () use ($app) {
if (!is_readable($configFile = $app['microrest.config_file'])) {
throw new \RuntimeException("API config file is not readable");
}
$apiDefinition = (new Parser())->parse($configFile);
$app['microrest.mediaType'] = $apiDefinition->getDefaultMediaType() ?: 'application/json';
$app['microrest.routes'] = $apiDefinition
->getResourcesAsUri()
->getRoutes()
;
$baseUrl = $apiDefinition->getBaseUrl();
$app['microrest.api_url_prefix'] = $baseUrl ? substr(parse_url($baseUrl, PHP_URL_PATH), 1) : 'api';
$app['microrest.restController'] = $app->share(function () use ($app) {
return new RestController($app['db']);
});
$app['microrest.routeBuilder'] = $app->share(function () {
return new RouteBuilder();
});
$app['microrest.doc_url_prefix'] = 'doc';
$app['microrest.description'] = function () use ($apiDefinition) {
$resources = array();
foreach ($apiDefinition->getResources() as $resource) {
$endpoints = array();
foreach ($resource->getMethods() as $method) {
$endpoints[] = [
'uri' => $resource->getUri(),
'method' => $method->getType(),
'description' => $method->getDescription(),
];
}
foreach ($resource->getResources() as $endpoint) {
foreach ($endpoint->getMethods() as $method) {
$endpoints[] = [
'uri' => $endpoint->getUri(),
'method' => $method->getType(),
'description' => $method->getDescription(),
];
}
}
$resources[] = [
'uri' => $resource->getUri(),
'name' => $resource->getDisplayName(),
'description' => $resource->getDescription(),
'endpoints' => $endpoints,
];
}
return array(
'apiName' => $apiDefinition->getTitle(),
'resources' => $resources,
'baseUrl' => $apiDefinition->getBaseUrl(),
'version' => $apiDefinition->getVersion(),
);
};
$app['twig.loader.filesystem'] = $app->share($app->extend('twig.loader.filesystem', function ($loader, $app) {
$loader->addPath($app['microrest.templates_path'], 'Microrest');
return $loader;
}));
$app['microrest.templates_path'] = function () {
return __DIR__.'/../views';
};
});
$app['microrest.builder'] = function () use ($app) {
$app['microrest.initializer']();
$controllers = $app['microrest.routeBuilder']
->build($app['controllers_factory'], $app['microrest.routes'], 'microrest.restController');
$app['controllers']->mount($app['microrest.api_url_prefix'], $controllers);
$controllers = $app['controllers_factory'];
$controllers->get('/', function () use ($app) {
return $app['twig']->render('@Microrest/doc.html.twig', $app['microrest.description']);
});
$app['controllers']->mount($app['microrest.doc_url_prefix'], $controllers);
};
}
public function boot(Application $app)
{
$app['microrest.builder'];
}
}
<file_sep><?php
if (preg_match('#^/admin#', $_SERVER["REQUEST_URI"])) {
if ('/admin' === $_SERVER["REQUEST_URI"]) {
header("Location: /admin/");
exit;
}
return false; // serve the requested resource as-is.
}
use Symfony\Component\Debug\Debug;
require_once __DIR__.'/../vendor/autoload.php';
Debug::enable();
$app = require __DIR__.'/../src/app.php';
require __DIR__.'/../config/dev.php';
$app->run();
<file_sep><?php
namespace Marmelab\Microrest;
use Doctrine\DBAL\Connection;
use Pagerfanta\Adapter\DoctrineDbalAdapter;
use Pagerfanta\Pagerfanta;
use Symfony\Component\HttpFoundation\JsonResponse;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\HttpFoundation\Response;
class RestController
{
protected $dbal;
public function __construct(Connection $dbal)
{
$this->dbal = $dbal;
}
public function homeAction($availableRoutes)
{
return new JsonResponse($availableRoutes);
}
public function getListAction($objectType, Request $request)
{
$queryBuilder = $this->dbal
->createQueryBuilder()
->select('o.*')
->from($objectType, 'o')
;
if ($sort = $request->query->get('_sort')) {
$queryBuilder->orderBy($sort, $request->query->get('_sortDir', 'ASC'));
}
$countQueryBuilderModifier = function ($queryBuilder) {
$queryBuilder
->select('COUNT(DISTINCT o.id) AS total_results')
->setMaxResults(1)
;
};
$pager = new DoctrineDbalAdapter($queryBuilder, $countQueryBuilderModifier);
$nbResults = $pager->getNbResults();
$results = $pager->getSlice($request->query->get('_start', 0), $request->query->get('_end', 20));
return new JsonResponse($results, 200, array(
'X-Total-Count' => $nbResults,
));
}
public function postListAction($objectType, Request $request)
{
try {
$this->dbal->insert($objectType, $request->request->all());
} catch (\Exception $e) {
return new JsonResponse(array(
'errors' => array('detail' => $e->getMessage()),
), 400);
}
$id = (integer) $this->dbal->lastInsertId();
return $this->getObjectResponse($objectType, $id, 201);
}
public function getObjectAction($objectId, $objectType)
{
return $this->getObjectResponse($objectType, $objectId);
}
public function putObjectAction($objectId, $objectType, Request $request)
{
$data = $request->request->all();
$request->request->remove('id');
$result = $this->dbal->update($objectType, $data, array('id' => $objectId));
if (0 === $result) {
return new Response('', 404);
}
return $this->getObjectResponse($objectType, $objectId);
}
public function deleteObjectAction($objectId, $objectType)
{
$result = $this->dbal->delete($objectType, array('id' => $objectId));
if (0 === $result) {
return new Response('', 404);
}
return new JsonResponse('', 204);
}
private function getObjectResponse($name, $id, $status = 200)
{
$queryBuilder = $this->dbal->createQueryBuilder();
$query = $queryBuilder
->select('*')
->from($name)
->where('id = '.$queryBuilder->createPositionalParameter($id))
;
$result = $query->execute()->fetchObject();
if (false === $result) {
return new Response('', 404);
}
return new JsonResponse($result, $status);
}
}
<file_sep><?php
namespace Marmelab\MicrorestTest;
use Marmelab\Microrest\MicrorestServiceProvider;
use Silex\Application;
use Symfony\Component\HttpFoundation\Request;
class MicrorestServiceProviderTest extends \PHPUnit_Extensions_Database_TestCase
{
private $connection = null;
/**
* @return PHPUnit_Extensions_Database_DB_IDatabaseConnection
*/
public function getConnection()
{
$databasePath = __DIR__ . "/Fixtures/api.db";
$config = new \Doctrine\DBAL\Configuration();
$connectionParams = array(
'path' => $databasePath,
'driver' => 'pdo_sqlite',
);
$this->connection = \Doctrine\DBAL\DriverManager::getConnection($connectionParams, $config);
return $this->createDefaultDBConnection($this->connection->getWrappedConnection(), 'api');
}
/**
* @return PHPUnit_Extensions_Database_DataSet_IDataSet
*/
public function getDataSet()
{
return new \PHPUnit_Extensions_Database_DataSet_YamlDataSet(
dirname(__FILE__)."/Fixtures/data.yml"
);
}
public function testSimpleRamlApiFile()
{
$app = new Application();
$app['debug'] = true;
$app->register(new \Silex\Provider\DoctrineServiceProvider(), array(
'db.options' => array(
'driver' => 'pdo_sqlite',
'path' => __DIR__ . "/Fixtures/api.db",
),
));
$app->register(new \Silex\Provider\ServiceControllerServiceProvider());
$app->register(new \Silex\Provider\TwigServiceProvider());
$app->register(new MicrorestServiceProvider(), array(
'microrest.config_file' => __DIR__.'/Fixtures/api.rml',
));
$request = Request::create('/api/artists', 'GET');
$response = $app->handle($request);
$this->assertEquals('[{"id":"1","name":"<NAME>","description":"French electronic music duo consisting of musicians <NAME> and <NAME>","image_url":"http:\/\/travelhymns.com\/wp-content\/uploads\/2013\/06\/random-access-memories1.jpg","nationality":"France"},{"id":"2","name":"<NAME>","description":"English rock band that achieved international acclaim with their progressive and psychedelic music.","image_url":"http:\/\/www.billboard.com\/files\/styles\/promo_650\/public\/stylus\/1251869-pink-floyd-reunions-617-409.jpg","nationality":"England"},{"id":"3","name":"Radiohead","description":"English rock band from Abingdon, Oxfordshire, formed in 1985","image_url":"http:\/\/www.billboard.com\/files\/styles\/promo_650\/public\/stylus\/1251869-pink-floyd-reunions-617-409.jpg","nationality":"England"}]', $response->getContent());
}
}
<file_sep><?php
use JDesrosiers\Silex\Provider\CorsServiceProvider;
use Marmelab\Microrest\MicrorestServiceProvider;
use Silex\Application;
use Silex\Provider\ServiceControllerServiceProvider;
use Silex\Provider\TwigServiceProvider;
use Silex\Provider\UrlGeneratorServiceProvider;
use Silex\Provider\ValidatorServiceProvider;
$app = new Application();
$app->register(new UrlGeneratorServiceProvider());
$app->register(new ValidatorServiceProvider());
$app->register(new ServiceControllerServiceProvider());
$app->register(new MicrorestServiceProvider(), array(
'microrest.config_file' => __DIR__ . '/../config/api/api.raml',
));
$app->register(new TwigServiceProvider(), array(
'twig.options' => array('cache' => __DIR__.'/../var/cache/twig'),
));
$app->register(new CorsServiceProvider(), array(
'cors.allowOrigin' => 'http://localhost:8000',
'cors.exposeHeaders' => 'X-Total-Count',
));
$app->after($app['cors']);
return $app;
<file_sep>Microrest.php demo
==================
The demo use ng-admin backend adminstration application.
Installation
------------
make install-demo
make run-demo<file_sep><?php
namespace Marmelab\Microrest;
use Silex\Application;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\HttpFoundation\Response;
class RouteBuilder
{
private static $validMethods = array('GET', 'POST', 'PUT', 'PATCH', 'DELETE');
public function build($controllers, array $routes, $controllerService)
{
$availableRoutes = array();
$beforeMiddleware = function (Request $request, Application $app) {
if (0 === strpos($request->headers->get('Content-Type'), $app['microrest.mediaType'])) {
$data = json_decode($request->getContent(), true);
$request->request->replace(is_array($data) ? $data : array());
}
};
$afterMiddleware = function (Request $request, Response $response, Application $app) {
$response->headers->set('Content-Type', $app['microrest.mediaType']);
};
foreach ($routes as $index => $route) {
$route['method'] = $route['type'];
unset($route['type']);
if (!in_array($route['method'], self::$validMethods)) {
continue;
}
$availableRoutes[] = $index;
if (preg_match('/{[\w-]+}/', $route['path'], $identifier)) {
$route['type'] = 'Object';
$route['objectType'] = strtolower(str_replace(array('/', $identifier[0]), '', $route['path']));
$route['path'] = str_replace($identifier[0], '{objectId}', $route['path']);
} else {
$route['type'] = 'List';
$route['objectType'] = strtolower(str_replace('/', '', $route['path']));
}
$action = $controllerService.':'.strtolower($route['method']).$route['type'].'Action';
$name = 'microrest.'.strtolower($route['method']).ucfirst($route['objectType']).$route['type'];
$controllers
->match($route['path'], $action)
->method($route['method'])
->setDefault('objectType', $route['objectType'])
->bind($name)
->before($beforeMiddleware)
->after($afterMiddleware)
;
}
$controllers->match('/', $controllerService.':homeAction')
->method('GET')
->setDefault('availableRoutes', $availableRoutes)
->bind('microrest.home')
->after($afterMiddleware)
;
return $controllers;
}
}
<file_sep><table>
<tr>
<td><img width="20" src="https://cdnjs.cloudflare.com/ajax/libs/octicons/8.5.0/svg/archive.svg" alt="archived" /></td>
<td><strong>Archived Repository</strong><br />
This code is no longer maintained. Feel free to fork it, but use it at your own risks.
</td>
</tr>
</table>
# Marmelab Microrest
Microrest is a Silex provider to setting up a REST API on top of a relational database, based on a YAML (RAML) configuration file.
Check out the [launch post](http://marmelab.com/blog/2015/01/05/introducing-microrest-raml-api-in-silex.html).
## What is RAML ?
[RESTful API Modeling Language (RAML)](http://raml.org/) is a simple and succinct way of describing practically-RESTful APIs. It encourages reuse, enables discovery and pattern-sharing, and aims for merit-based emergence of best practices.
You can easily set up a RAML file from [API Designer](http://api-portal.anypoint.mulesoft.com/raml/api-designer).
## Installation
To install microrest.php library, run the command below and you will get the latest version:
```bash
composer require marmelab/microrest "~1.0@dev"
```
Enable `ServiceController`, `Doctrine` and `Microrest` service providers in your application:
```php
$app->register(new Silex\Provider\ServiceControllerServiceProvider());
$app->register(new Silex\Provider\DoctrineServiceProvider(), array(
'db.options' => array(
'driver' => 'pdo_sqlite',
'path' => __DIR__.'/app.db',
),
));
$app->register(new Marmelab\Microrest\MicrorestServiceProvider(), array(
'microrest.config_file' => __DIR__ . '/api.raml',
));
```
You need to give the path to the `RAML` file describing your API. You can find an example into the `tests/fixtures` directory.
Then, browse your new API REST on the url defined in the `baseUrl` configuration of your `RAML` api file.
## Tests
Run the tests suite with the following commands:
```bash
make install
make test
```
## Demo
You can find a complete demo application in `examples/ng-admin`. You just need 2 commands to install and run it:
```bash
make install-demo
make run-demo
```
Play with the Silex demo API at the url: `http://localhost:8888/api`
Explore the API using [ng-admin](https://github.com/marmelab/ng-admin) backend administration at the url: `http://localhost:8888/admin`
## License
microrest.php is licensed under the [MIT License](LICENSE), courtesy of [marmelab](http://marmelab.com).
<file_sep><?php
$app->register(new Silex\Provider\DoctrineServiceProvider(), array(
'db.options' => array(
'driver' => 'pdo_sqlite',
'path' => __DIR__.'/../app.db',
),
));<file_sep>.PHONY: test
install-demo:
composer -d=examples/ng-admin install
bower --config.cwd=./examples/ng-admin/web/admin install
cp examples/ng-admin/app.db-dist examples/ng-admin/app.db
run-demo:
composer -d=examples/ng-admin run
install:
composer install --dev --prefer-source
test:
composer test
| 533befc3c6e9d74bdcb4f8577520d83409af5e2a | [
"Markdown",
"Makefile",
"PHP"
] | 10 | PHP | alexisjanvier/microrest.php | c9e0e951c42e69da1635b1e2de449e367d4edfaa | e5f5bfc7d4e60178e2884af2ae649b5eb26e176d | |
refs/heads/main | <file_sep>/**
* // This is the robot's control interface.
* // You should not implement it, or speculate about its implementation
* interface Robot {
* // Returns true if the cell in front is open and robot moves into the cell.
* // Returns false if the cell in front is blocked and robot stays in the current cell.
* public bool Move();
*
* // Robot will stay in the same cell after calling turnLeft/turnRight.
* // Each turn will be 90 degrees.
* public void TurnLeft();
* public void TurnRight();
*
* // Clean the current cell.
* public void Clean();
* }
*/
class Solution {
Robot r;
HashSet<ValueTuple<int,int>> visited;
public void CleanRoom(Robot robot) {
r = robot;
visited = new HashSet<ValueTuple<int,int>>();
BackTrack((0, 0), Direction.Up);
}
private void BackTrack(ValueTuple<int, int> pos, Direction d){
visited.Add(pos);
r.Clean();
for(var i = 0; i < 4; i++){
var newPos = GetPos(pos, d);
if(!visited.Contains(newPos) && r.Move()){
BackTrack(newPos, d);
}
r.TurnRight();
d = (Direction)(((int)d + 1)%4);
}
GoBack();
}
public void GoBack() {
r.TurnRight();
r.TurnRight();
r.Move();
r.TurnRight();
r.TurnRight();
}
private ValueTuple<int, int> GetPos(ValueTuple<int, int> pos, Direction d){
(var row, var col) = pos;
switch(d){
case Direction.Up:
return (row-1, col);
case Direction.Right:
return (row, col+1);
case Direction.Down:
return (row+1, col);
case Direction.Left:
return (row, col-1);
}
return pos;
}
private enum Direction{
Up,
Right,
Down,
Left
}
}<file_sep>public class Solution {
public IList<IList<int>> Subsets(int[] nums) {
var subsets = new List<IList<int>>();
subsets.Add(new List<int>());
// cascading - copy previous subsets 2^N
// []
// [] [1]
// [] [2] [1, 2]
for(int i = 0; i < nums.Length; i++){
for(int j = subsets.Count-1; j >= 0; j--){
var set = new List<int>(subsets[j]);
set.Add(nums[i]);
subsets.Add(set);
}
}
return subsets;
}
// OR backtrack
public IList<IList<int>> Subsets(int[] nums) {
var res = new List<IList<int>>();
for(int setSize = 0; setSize <= nums.Length; setSize++)
BackTrack(nums, 0, setSize, new List<int>(), res);
return res;
}
void BackTrack(int[] nums, int start, int setSize, List<int> currentSet, IList<IList<int>> res){
if(currentSet.Count == setSize){
res.Add(new List<int>(currentSet));
return;
}
for(int i = start; i < nums.Length; i++){
currentSet.Add(nums[i]);
BackTrack(nums, i+1, setSize, currentSet, res);
currentSet.RemoveAt(currentSet.Count-1);
}
}
}<file_sep>public class Solution {
public int[][] Insert(int[][] intervals, int[] newInterval) {
var n = intervals.Length;
var res = new List<int[]>();
var ptr = 0;
while (ptr < n && intervals[ptr][1] < newInterval[0]){
res.Add(intervals[ptr++]);
}
var i = new int[]{newInterval[0], newInterval[1]};
res.Add(i);
while (ptr < n){
var next = intervals[ptr++];
if (i[1] >= next[0]){
i[0] = Math.Min(i[0], next[0]);
i[1] = Math.Max(i[1], next[1]);
} else {
res.Add(next);
}
}
return res.ToArray();
}
}<file_sep>/**
* Definition for singly-linked list.
* public class ListNode {
* public int val;
* public ListNode next;
* public ListNode(int val=0, ListNode next=null) {
* this.val = val;
* this.next = next;
* }
* }
*/
public class Solution {
public ListNode MergeKLists(ListNode[] lists) {
if(lists == null || lists.Length == 0)
return null;
var n = lists.Length;
if (n == 1)
return lists[0];
var step = 1;
if(n % 2 != 0){
lists[0] = MergeTwo(lists[0], lists[n-1]);
n--;
}
while(step < n){
for(var i = 0; i < n-step; i += step*2){
lists[i] = MergeTwo(lists[i], lists[i+step]);
}
step *= 2;
}
return lists[0];
}
private ListNode MergeTwo(ListNode l1, ListNode l2){
var dummy = new ListNode();
var head = dummy;
while(l1 != null && l2 != null){
if(l1.val < l2.val){
head.next = l1;
l1 = l1.next;
} else {
head.next = l2;
l2 = l2.next;
}
head = head.next;
}
var l = l1 == null ? l2 : l1;
if(l != null)
head.next = l;
return dummy.next;
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public IList<TreeNode> GenerateTrees(int n) {
if(n==0)
return new List<TreeNode>();
return GenerateTrees(1, n);
}
public IList<TreeNode> GenerateTrees(int start, int end) {
var trees = new List<TreeNode>();
if (start > end){
trees.Add(null);
return trees;
}
for(int i = start; i <=end; i++){
var lTrees = GenerateTrees(start, i-1);
var rTrees = GenerateTrees(i+1, end);
foreach(var l in lTrees)
foreach(var r in rTrees){
trees.Add(new TreeNode(i, l, r));
}
}
return trees;
}
}<file_sep>// morris traversal
public class Solution {
public void RecoverTree(TreeNode root) {
TreeNode prev = null;
TreeNode firstNode = null;
TreeNode secondNode = null;
void check(TreeNode node){
if(prev != null && node.val < prev.val){
if(firstNode == null)
firstNode = prev;
secondNode = node;
}
prev = node;
}
var cur = root;
while(cur != null){
if(cur.left == null){
check(cur);
cur = cur.right;
} else {
var pred = cur.left;
while(pred.right != cur && pred.right != null)
pred = pred.right;
if(pred.right == null){ // not visited
pred.right = cur;
// visit pre-order
cur = cur.left;
} else { // visited
pred.right = null;
check(cur); // in-order
cur = cur.right;
}
}
}
var t = firstNode.val;
firstNode.val = secondNode.val;
secondNode.val = t;
}
}
public class Solution {
public void RecoverTree(TreeNode root) {
var st = new Stack<TreeNode>();
void traverseLeft(TreeNode node){
while(node != null){
st.Push(node);
node = node.left;
}
}
traverseLeft(root);
TreeNode prev = null;
TreeNode firstNode = null;
TreeNode secondNode = null;
while(st.Any()){
var node = st.Pop();
if(prev != null && node.val < prev.val){
if(firstNode == null)
firstNode = prev;
secondNode = node;
}
prev = node;
traverseLeft(node.right);
}
var t = firstNode.val;
firstNode.val = secondNode.val;
secondNode.val = t;
}
}<file_sep>public class Solution {
public string Multiply(string num1, string num2) {
var n = num1.Length;
var m = num2.Length;
var res = new int[n+m];
for(var i=n-1; i>=0; i--){
for(var j = m-1; j>=0; j--){
var mult = (num1[i]-'0') * (num2[j]-'0');
var p1 = i+j;
var p2 = p1+1;
var sum = res[p2] + mult;
res[p2] = sum % 10;
res[p1] += sum / 10;
}
}
var pos = 0;
while(res[pos] == 0){
if (pos == res.Length-1)
return "0";
pos++;
}
return new String(res[pos..].Select(c => (char)(c+'0')).ToArray());
}
}<file_sep>public class Solution {
public int MinCost(int[][] grid) {
var rows = grid.Length;
var cols = grid[0].Length;
if(rows == 0 && cols == 0)
return 0;
var dirs = new (int,int)[]{(0,1),(0,-1),(1,0),(-1,0)};
var map = new SortedDictionary<int, Queue<(int, int)>>();
var dist = new int?[rows, cols];
map.Add(0, new Queue<(int, int)>());
map[0].Enqueue((0,0));
dist[0,0] = 0;
while(map.Any()){
var node = map.First();
var cost = node.Key;
var (row, col) = node.Value.Dequeue();
if(!node.Value.Any())
map.Remove(cost);
for(int i = 0; i < 4; i++){
var (rd,cd) = dirs[i];
var r = row+rd;
var c = col+cd;
var newCost = cost + (i == grid[row][col]-1 ? 0 : 1);
if(r >= 0 && r < rows && c >= 0 && c < cols && (dist[r,c] == null || dist[r,c] > newCost)){
dist[r,c] = newCost;
if(!map.ContainsKey(newCost))
map.Add(newCost, new Queue<(int, int)>());
map[newCost].Enqueue((r,c));
}
}
}
return dist[rows-1, cols-1].Value;
}
} <file_sep>public class Solution {
public int MaxSumTwoNoOverlap(int[] A, int L, int M) {
var n = A.Length;
var maxSoFar = new int[n,2];
var res = -1;
var sumL = 0;
var maxL = int.MinValue;
var sumM = 0;
var maxM = int.MinValue;
for(int hi = 0; hi <n; hi++){
sumL += A[hi];
sumM += A[hi];
if(hi-L >= -1){
if (hi-L > -1)
sumL -= A[hi-L];
maxL = Math.Max(maxL, sumL);
maxSoFar[hi,0] = maxL;
if (hi-L > -1)
res = Math.Max(res, sumL + maxSoFar[hi-L, 1]);
}
if(hi-M >= -1){
if (hi-M > -1)
sumM -= A[hi-M];
maxM = Math.Max(maxM, sumM);
maxSoFar[hi,1] = maxM;
if (hi-M > -1)
res = Math.Max(res, sumM + maxSoFar[hi-M, 0]);
}
//Console.WriteLine(maxL + " " + maxM);
}
return res;
}
}<file_sep>public class Solution {
public bool Exist(char[][] board, string word) {
if(word == null || word == "")
return false;
var rows = board.Length;
var cols = board[0].Length;
var delta = new []{(0,1),(1,0),(0,-1),(-1,0)};
for(int r = 0; r < rows; r++){
for(int c = 0; c < cols; c++){
if(DFS(r, c, 0, new HashSet<(int, int)>()))
return true;
}
}
bool DFS(int row, int col, int pos, HashSet<(int, int)> visited){
if(pos >= word.Length)
return true;
if(row < 0 || row >= rows || col < 0 || col >= cols || board[row][col] != word[pos] || visited.Contains((row, col)))
return false;
visited.Add((row, col));
var found = false;
foreach(var (rd, cd) in delta){
if(found = DFS(row + rd, col + cd, pos+1, visited))
break;
}
visited.Remove((row, col));
return found;
}
return false;
}
}<file_sep>public class Solution {
public int LadderLength(string beginWord, string endWord, IList<string> wordList) {
var graph = new Dictionary<string, HashSet<string>>();
var wl = wordList;
var len = wl[0].Length;
bool isNext(string a, string b){
var count = 0;
for(var c = 0; c < len && count < 2; c++){
if(a[c] != b[c])
count++;
}
return count==1;
}
var hasEnd = false;
for(var i = -1; i< wl.Count; i++){
var word = i == -1 ? beginWord : wl[i];
var node = graph[word] = new HashSet<string>();
hasEnd = hasEnd || word == endWord;
for(var j = 0; j < wl.Count; j++){
if(isNext(word,wl[j]))
node.Add(wl[j]);
}
}
if(!hasEnd || beginWord == endWord)
return 0;
var min = int.MaxValue;
var step = 1;
var q = new Queue<string>();
var visited = new HashSet<string>{beginWord};
q.Enqueue(beginWord);
while(q.Count > 0 ){
for(var i = q.Count; i > 0; i--){
var w = q.Dequeue();
if(w == endWord){
min = Math.Min(min, step);
continue;
}
foreach(var child in graph[w]){
if(!visited.Contains(child)){
q.Enqueue(child);
visited.Add(child);
}
}
}
step++;
}
return min == int.MaxValue ? 0 : min;
}
}<file_sep>public class Solution {
public int CombinationSum4(int[] nums, int target) {
var n = nums.Length;
int dfs(int sum, Dictionary<int, int> memo){
if(sum > target)
return 0;
if(sum == target)
return 1;
int res = 0;
if(memo.TryGetValue(sum, out res))
return res;
foreach(var num in nums)
res += dfs(sum+num, memo);
memo[sum] = res;
return res;
}
return dfs(0, new Dictionary<int,int>());
}
}<file_sep>public class Solution {
public int ClimbStairs(int n) {
//var steps = new int[n];
var prev1 = 2;
var prev2 = 1;
for(int i = 2; i < n; i++){
var curr = prev1 + prev2;
prev2 = prev1;
prev1 = curr;
}
return n > 1 ? prev1 : prev2;
}
}<file_sep>public class Solution {
/*
o,1 2,0 3,4
0 1 2 3 4
1 1 1 3 4
*/
public int CountComponents(int n, int[][] edges) {
var dsu = new DSU(n);
foreach(var edge in edges){
dsu.Union(edge[0], edge[1]); // 2 0
}
/*
var res = 0;
for(int i = 0; i < n; i++){
if(dsu.nodes[i] == i)
res++;
}
*/
return dsu.n;
}
public class DSU{
public int n;
public int[] nodes;
public DSU(int n){
this.n = n;
nodes = Enumerable.Range(0, n).ToArray();
}
public void Union(int a, int b){
// 2
// 1
var pa = Find(a);
var pb = Find(b);
if(pa != pb){
nodes[pa] = pb;
n--;
}
}
public int Find(int a){
if(nodes[a] != a)
nodes[a] = Find(nodes[a]); // 1
return nodes[a];
}
}
}<file_sep>public class Solution {
public bool IsMonotonic(int[] A) {
var isIncreasing = 0;
for(int i = 1; i < A.Length; i++){
if(isIncreasing == 0){
isIncreasing = A[i] - A[i-1];
} else {
if(isIncreasing * (A[i] - A[i-1]) < 0)
return false;
}
}
return true;
}
}<file_sep>using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
namespace Solution {
class Solution {
static void Main(string[] args) {
/* Enter your code here. Read input from STDIN. Print output to STDOUT */
/*
1, 3, b
2, 3, c
3,null, a -
5,2, d
1,3
2,3
count: 3, 1 ,2 5
count: (2, a), (1,b), (3, a+b)
map: (2, 3), (1, 3), (3, 3)
{
paretid,
id,
count
}
(3,3, ) (3,1) (3,2)*/
}
public (int,int)[] TopBrands(int k, (int, int?, int)[] brandInfos){
var dsu = new DSU();
foreach(var info in brandInfos){
dsu.Union(info);
}
var counts = new Dictionary<int, int>();
foreach(var kv in dsu.Counts){
if (kv.Key = dsu.Find(kv.Key))
counts[kv.Key] = kv.Value;
}
var minHeap = new Heap<(int, int)>((a, b)=> a.Count - b.Count);
/*foreaach(var kv in Counts){
if(minHeap.Count >= k && minHeap.Top.Count < kv.Value)
minHeap.RemoveTop();
if(minHeap.Count < k)
minHeap.Push(kv)
}*/
return minHeap.ToArray();
}
}
// Xa,
// 1,2,3,4,5,6
// 1
// 2,3,4
class DSU{
public Dictionary<int, int> map = new Dictionary<int, int>();
public Dictionary<int, count> counts = new Dictionary<int, int>();
public void Union(int id, int? parentId, int count){
if(parentId == null){
map[id] = id;
counts[id] = count;
} else {
// todo when parent weas not found
var p1 = Find(parentId);
var p2 = Find(id);
map[p1] = p2;
count[p1] += count;
}
}
public int Find(int id){
if(!map.ContainsKey(id)){
map[id] = id;
counts[id] = 0;
}
if(map[id] == id)
return id;
return Find(map[id]);
}
}
}
<file_sep>public class Solution {
public int LeastInterval(char[] tasks, int n) {
var count = tasks.Length;
var frq = new int[26];
var max = 0;
var maxCount = 1;
for(int i = 0; i < count; i++){
var idx = tasks[i]-'A';
frq[idx]++;
if(frq[idx] > max){
max = frq[idx];
maxCount = 1;
} else if(frq[idx] == max){
maxCount++;
}
}
var minStepsToScheduleMax = (max-1)*(n+1);
/*
n+1 cols
xxxxxx max-1 rows
xxxxxx
xxxxxx
x appendix number of max frqs
*/
return Math.Max(count, minStepsToScheduleMax+maxCount);
}
}<file_sep>/*
// Definition for a Node.
public class Node {
public int val;
public Node left;
public Node right;
public Node parent;
}
*/
public class Solution {
public Node LowestCommonAncestor(Node p, Node q) {
var path = new HashSet<Node>();
while(p != null){
path.Add(p);
p = p.parent;
}
while(q != null){
if(path.Contains(p))
return p;
p = p.parent;
}
return null;
}
}<file_sep>public class Solution {
public bool CanConvert(string str1, string str2) {
var n = str1.Length;
var dsu = new DSU();
var loops = 0;
for(var i = 0; i < n; i++){
var a = str1[i];
var b = str2[i];
if(a == b)
continue;
if(dsu.HasAnotherChild(a, b))
return false;
if(!dsu.Union(a, b))
loops++;
}
var jokers = 26 - (new HashSet<char>(str2).Count);
return jokers >= loops;
}
class DSU{
public Dictionary<char, Node> map = new Dictionary<char, Node>();
public bool HasAnotherChild(char a, char b){
return map.ContainsKey(a) && map[a].Child != null && map[a].Child.Val != b;
}
public bool Union(char a, char b){
Node nodeA = FindOrCreate(a);
Node nodeB = FindOrCreate(b);
if(nodeB.Parent == nodeA)
return true;
Node parentA = nodeA;
Node parentB = nodeB;
while(parentA.Parent != parentA)
parentA = parentA.Parent;
while(parentB.Parent != parentB)
parentB = parentB.Parent;
if(parentA == parentB)
return false;
nodeB.Parent = nodeA;
nodeA.Child = nodeB;
return true;
}
private Node FindOrCreate(char c){
if(map.ContainsKey(c))
return map[c];
else{
var n = new Node(c);
map[c] = n;
return n;
}
}
}
class Node{
public char Val;
public Node Parent;
public Node Child;
public Node(char val){
Val = val;
Parent = this;
}
}
}<file_sep>public class Solution {
public int MaximalSquare(char[][] matrix) {
var r = matrix.Length;
if (r==0)
return 0;
var c = matrix[0].Length;
var dp = new int[r+1, c+1];
var maxSquare = 0;
for(int i=1; i<=r; i++){
for(int j =1; j<=c; j++){
if(matrix[i-1][j-1] == '1'){
dp[i,j]=Math.Min(Math.Min(dp[i-1,j],dp[i,j-1]), dp[i-1,j-1])+1;
if (dp[i,j] > maxSquare)
maxSquare = dp[i,j];
}
}
}
return maxSquare*maxSquare;
}
}<file_sep>public class Solution {
public int MaxSubArray(int[] nums) {
var localSum = 0;
var maxSum = int.MinValue;
foreach(var n in nums){
localSum += n;
maxSum = Math.Max(localSum, maxSum);
localSum = Math.Max(0, localSum);
}
return maxSum;
}
}<file_sep>public class LFUCache {
Dictionary<int, LinkedListNode<CacheNode>> nodeMap;
Dictionary<int, LinkedList<CacheNode>> countMap;
int capacity;
int length;
int minCount;
public LFUCache(int capacity) {
this.capacity = capacity;
nodeMap = new Dictionary<int, LinkedListNode<CacheNode>>();
countMap = new Dictionary<int, LinkedList<CacheNode>>();
}
public int Get(int key) {
if(nodeMap.TryGetValue(key, out var node)){
Update(node);
return node.Value.Val;
}
return -1;
}
public void Put(int key, int value) {
if(capacity == 0)
return;
// override key
if(nodeMap.TryGetValue(key, out var node)){
node.Value.Val = value;
Update(node);
} else { // new key
length++;
// invalidate
if(length > capacity){
nodeMap.Remove(countMap[minCount].First.Value.Key);
countMap[minCount].RemoveFirst();
length--;
}
// new cache node
nodeMap[key] = AddCacheNode(new CacheNode(key, value));
minCount = 1;
}
}
private void Update(LinkedListNode<CacheNode> node){
var cacheNode = node.Value;
// moving last node of min count
if(node.List.Count == 1 && cacheNode.Count == minCount)
minCount++;
node.List.Remove(node);
cacheNode.Count++;
nodeMap[cacheNode.Key] = AddCacheNode(cacheNode);
}
// add node to count map
private LinkedListNode<CacheNode> AddCacheNode(CacheNode cacheNode){
if(!countMap.ContainsKey(cacheNode.Count))
countMap[cacheNode.Count] = new LinkedList<CacheNode>();
countMap[cacheNode.Count].AddLast(cacheNode);
return countMap[cacheNode.Count].Last;
}
class CacheNode{
public int Key;
public int Val;
public int Count;
public CacheNode(int key, int val){
Key = key;
Val = val;
Count = 1;
}
}
}<file_sep>public class Solution {
public bool CheckSubarraySum(int[] nums, int k) {
var map = new Dictionary<int, int>{{0, -1}};
var sum = 0;
for(int i = 0; i< nums.Length; i++){
sum += nums[i];
if(k != 0){
var mod = (sum % k);
if(map.ContainsKey(mod)){
if(i - map[mod] >= 2)
return true;
}
else{
map.Add(mod, i);
}
} else {
if(i > 0 && nums[i] == 0 && nums[i-1] == nums[i])
return true;
}
}
return false;
}
}<file_sep>public class Solution {
public int FindMaxForm(string[] strs, int m, int n) {
var len = strs.Length;
var memo = new int?[len, m, n];
var res = int.MinValue;
int DFS(int pos, int mCurr, int nCurr){
if(pos >= len)
return 0;
if(memo[pos, mCurr, nCurr] != null)
return memo[pos].Value;
var str = strs[pos];
var mt = 0;
var nt = 0;
foreach(var c in str){
if(c == '0')
mt++;
else
nt++;
}
count = DFS(pos+1, mCurr, nCurr);
if(mCurr+mt <= m && nCurr+nt <=n){
count = Math.Max(count, DFS(pos+1, mCurr+mt, nCurr+nt)+1);
}
memo[pos, mCurr, nCurr] = count;
return count;
}
DFS(0, 0, 0);
return memo[0, 0, 0];
}
}<file_sep>public class Solution {
public IList<string> RemoveComments(string[] source) {
var res = new List<string>();
var inBlock = false;
StringBuilder sb = null;
foreach(var l in source){
if(!inBlock)
sb = new StringBuilder();
var n = l.Length;
var starIndex = -1;
for(int i=0; i < n; i++){
if(!inBlock){
if(l[i] == '/' && i < n-1){
if(l[i+1]=='/')
break;
else if(l[i+1]=='*'){
inBlock = true;
starIndex = i+1;
} else {
sb.Append(l[i]);
}
} else {
sb.Append(l[i]);
}
} else if(i > 0 && l[i] == '/' && l[i-1] == '*' && i-1 != starIndex) { // /*/ - not valid end of block
inBlock = false;
}
}
if(!inBlock && sb.Length > 0)
res.Add(sb.ToString());
}
return res;
}
}<file_sep>public class Solution {
public bool IsSubtree(TreeNode s, TreeNode t) {
var sTree = Preorder(s);
var tTree = Preorder(t);
return sTree.IndexOf(tTree) != -1;
}
string Preorder(TreeNode node){
return node == null
? "n"
: string.Concat("#", node.val, Preorder(node.left), Preorder(node.right));
}
}<file_sep>public class Solution {
public IList<IList<int>> Generate(int numRows) {
var res = new List<IList<int>>();
for(int i = 0; i < numRows; i++){
var row = new List<int>();
res.Add(row);
for(int j = 0; j <= i; j++){
if(j == 0 || j == i){
row.Add(1);
} else{
row.Add(res[i-1][j-1]+res[i-1][j]);
}
}
}
return res;
}
}<file_sep>public class Solution {
public int MaxProduct(int[] nums) {
int max = int.MinValue;
int product=1;
foreach(int num in nums){
product *= num;
max = Math.Max(product,max);
if(product == 0)product = 1;
}
product = 1;
for(int i=nums.Length-1;i>=0;i--){
product *= nums[i];
max = Math.Max(product,max);
if(product ==0) product = 1;
}
return max;
}
}<file_sep>/**
* Definition for singly-linked list.
* public class ListNode {
* public int val;
* public ListNode next;
* public ListNode(int val=0, ListNode next=null) {
* this.val = val;
* this.next = next;
* }
* }
*/
public class Solution {
public ListNode AddTwoNumbers(ListNode l1, ListNode l2) {
return GetSum(l1, l2, 0);
}
public ListNode GetSum(ListNode l1, ListNode l2, int carry){
if (l1 == null && l2 == null)
return carry > 0 ? new ListNode(carry) : null;
var sum = (l1?.val ?? 0) + (l2?.val ?? 0) + carry;
var res = new ListNode(sum % 10);
res.next = GetSum(l1?.next, l2?.next, sum / 10);
return res;
}
}<file_sep>public class Solution {
public int NumSquares(int n) {
var dp = Enumerable.Repeat(int.MaxValue, n+1).ToArray();
dp[0] = 0;
for(int i = 1; i <= n; i++){
for(int j = 1; j*j <= i; j++){
dp[i] = Math.Min(dp[i], dp[i - j*j] + 1);
}
}
return dp[n];
}
}<file_sep>public class TicTacToe {
int[] rows;
int[] cols;
int d1;
int d2;
int n;
/** Initialize your data structure here. */
public TicTacToe(int n) {
rows = new int[n];
cols = new int[n];
this.n = n;
}
/** Player {player} makes a move at ({row}, {col}).
@param row The row of the board.
@param col The column of the board.
@param player The player, can be either 1 or 2.
@return The current winning condition, can be either:
0: No one wins.
1: Player 1 wins.
2: Player 2 wins. */
public int Move(int row, int col, int player) {
var p = player == 1 ? 1 : -1;
rows[row] += p;
cols[col] += p;
if(row == col)
d1 += p;
if(row + col == n-1)
d2 += p;
return (Math.Abs(rows[row]) == n || Math.Abs(cols[col]) == n || Math.Abs(d1) == n || Math.Abs(d2) == n) ? player : 0;
}
}
/**
* Your TicTacToe object will be instantiated and called as such:
* TicTacToe obj = new TicTacToe(n);
* int param_1 = obj.Move(row,col,player);
*/<file_sep>public class Solution {
public int[][] GenerateMatrix(int n) {
var val = 1;
var res = new int[n][];
for(int i = 0; i < n; i++)
res[i] = new int[n];
var top = 0;
var bottom = n-1;
var left = 0;
var right = n-1;
while(val <= n*n){
for(int c = left; c <= right; c++)
res[top][c] = val++;
top++;
for(int r = top; r <= bottom; r++)
res[r][right] = val++;
right--;
for(int c = right; c >= left; c--)
res[bottom][c] = val++;
bottom--;
for(int r = bottom; r >= top; r--)
res[r][left] = val++;
left++;
}
return res;
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public int GoodNodes(TreeNode root) {
var res = 0;
GoodNodes(root, int.MinValue, ref res);
return res;
}
void GoodNodes(TreeNode node, int max, ref int res){
if(node == null)
return;
if(node.val >= max){
res++;
max = node.val;
}
GoodNodes(node.left, max, ref res);
GoodNodes(node.right, max, ref res);
}
}<file_sep>// 76, 3
public class Solution {
public IList<int> FindAnagrams(string s, string p) {
var res = new List<int>();
if(s.Length == 0 || p.Length > s.Length)
return res;
var frq = new Dictionary<char, int>();
foreach(var c in p)
frq[c] = frq.ContainsKey(c) ? frq[c] + 1 : 1;
var count = p.Length;
var lo = 0;
var hi = 0;
while(hi < s.Length){
var c = s[hi++];
if(frq.ContainsKey(c)){
frq[c]--;
if(frq[c] >= 0)
count--;
}
if(count == 0)
res.Add(lo);
if(hi-lo == p.Length){
c = s[lo++];
if(frq.ContainsKey(c)){
frq[c]++;
if(frq[c] > 0)
count++;
}
}
}
return res;
}
}<file_sep>public class MinStack {
private Stack<int> dataStack;
private Stack<int> minStack;
/** initialize your data structure here. */
public MinStack() {
dataStack = new Stack<int>();
minStack = new Stack<int>();
}
public void Push(int x) {
dataStack.Push(x);
if (x <= getMin())
minStack.Push(x);
}
public void Pop() {
var x = dataStack.Pop();
if (x == getMin())
minStack.Pop();
}
public int Top() {
return dataStack.Peek();
}
public int GetMin() {
return minStack.Peek();
}
private int getMin()
{
return minStack.Count == 0 ? int.MaxValue : minStack.Peek();
}
}
<file_sep>public class Solution {
public int FindKthPositive(int[] arr, int k) {
var lo = 0;
var hi = arr.Length-1;
while(lo <= hi){
var mid = lo+(hi-lo)/2;
if(arr[mid]-(mid+1) >= k)
hi = mid-1;
else
lo = mid+1;
}
return lo + k;
}
}<file_sep>public class Solution {
// 1994
// MCMXCIV
public string IntToRoman(int num) { //
var values = new []{1000,900,500,400,100,90,50,40,10,9,5,4,1};
var romans = new []{"M","CM","D","CD","C","XC","L","XL","X","IX","V","IV","I"};
var sb = new StringBuilder();
for(int i = 0; i < values.Length; i++){
while(num >= values[i]){
sb.Append(romans[i]);
num -= values[i];
}
}
return sb.ToString();
}
public string IntToRoman(int num) { //
var sb = new StringBuilder(); // MCMXC
var romans = new []{'M','D','C','L','X','V','I'};
var limit = 10000; // 100
var idx = -2; // 2 c
while(limit > 1){
var divisor = limit/10; // 10
if(num >= limit-divisor){ // 90
sb.Append(romans[idx+2]);
sb.Append(romans[idx]);
num -= limit-divisor; // 4
}
if(num >= limit/2){ // 500
sb.Append(romans[idx+1]);
num -= limit/2;
}
if(num >= limit/2 - divisor){
sb.Append(romans[idx+2]);
sb.Append(romans[idx+1]);
num -= limit/2 - divisor;
}
if(num >= divisor){
var count = num / divisor;
for(int i = 0; i < count; i++)
sb.Append(romans[idx+2]);
num -= divisor*count;
}
limit = divisor;
idx += 2;
}
return sb.ToString();
}
}<file_sep>public class Solution {
public int ConnectSticks(int[] sticks) {
var heap = new SortedDictionary<int,int>();
var count = 0;
foreach(var stick in sticks){
add(stick);
}
void add(int v){
count++;
if(heap.ContainsKey(v))
heap[v]++;
else
heap[v] = 1;
}
int remove(){
count--;
var v = heap.First().Key;
heap[v]--;
if(heap[v] == 0)
heap.Remove(v);
return v;
}
var res = 0;
while(count > 1){
var a = remove();
var b = remove();
add(a+b);
res += a+b;
}
return res;
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public int MaxPathSum(TreeNode root) {
var maxSum = int.MinValue;
MaxPathSum(root, ref maxSum);
return maxSum;
}
public int MaxPathSum(TreeNode root, ref int maxSum) {
if (root == null)
return 0;
var maxL = Math.Max(MaxPathSum(root.left, ref maxSum), 0);
var maxR = Math.Max(MaxPathSum(root.right, ref maxSum), 0);
maxSum = Math.Max(maxSum, maxL+maxR+root.val);
return Math.Max(maxL, maxR)+root.val;
}
}<file_sep>public class Solution {
public int[] SearchRange(int[] nums, int target) {
var leftIdx = Search(nums, target, 0, nums.Length, true);
if(leftIdx >= nums.Length || nums[leftIdx] != target)
return new[] {-1,-1};
return new[] {leftIdx, Search(nums, target, leftIdx, nums.Length, false)-1};
}
private int Search(int[] nums, int target, int lo, int hi, bool left){
while (lo < hi) {
int mid = (lo + hi) / 2;
if (nums[mid] > target || (left && target == nums[mid])) {
hi = mid;
}
else {
lo = mid+1;
}
}
return hi;
}
}<file_sep>public class Solution {
public int LengthOfLongestSubstringTwoDistinct(string s) {
if(s == null || s.Length <= 2)
return s?.Length ?? 0;
var lo = 0;
var hi = 0;
var max = 0;
var map = new Dictionary<char, int>();
while(hi < s.Length){
var c = s[hi++];
if(map.Count < 2 || map.ContainsKey(c)){
if(hi-lo > max)
max = hi - lo;
} else{
var prevVal = Math.Min(map.First().Value, map.Last().Value);
lo = prevVal;
map.Remove(s[prevVal-1]);
}
map[c] = hi;
}
return max;
}
}<file_sep>public class Solution {
public bool SearchMatrix(int[][] matrix, int target) {
var rows = matrix.Length;
if(rows == 0)
return false;
var cols = matrix[0].Length;
var n = rows * cols;
var lo = 0;
var hi = n-1;
while(lo <= hi){
var mid = lo + (hi-lo)/2;
var val = matrix[mid/cols][mid%cols];
if(target == val)
return true;
if(val > target)
hi = mid-1;
else
lo = mid+1;
}
return false;
}
}<file_sep>public class Solution {
public string BreakPalindrome(string palindrome) {
if(palindrome == null || palindrome.Length <=1)
return "";
var sb = palindrome.ToCharArray();
for(int i = 0; i < sb.Length/2; i++){
if(sb[i] != 'a'){
sb[i] = 'a';
return new string(sb);
}
}
sb[sb.Length-1] = 'b';
return new string(sb);
}
}<file_sep>public class Solution {
public string MinRemoveToMakeValid(string s) {
var n = s.Length;
var st = new Stack<int>();
var sb = new StringBuilder(s);
for(int i = 0; i < n; i++){
var c = sb[i];
if(c == '('){
st.Push(i);
} else if(c == ')'){
if(!st.Any())
sb[i] = '*';
else
st.Pop();
}
}
while(st.Any()){
sb[st.Pop()] = '*';
}
sb.Replace("*", "");
return sb.ToString();
}
}<file_sep>public class Solution {
public int CountSubstrings(string s) {
var palindroms = 0;
var fraim = 0;
while (fraim <= s.Length)
{
for (int i = fraim; i < s.Length; i++)
{
var end = i;
var start = i - fraim;
while (start <= end && s[start] == s[end])
{
start++;
end--;
}
if (start >= end)
palindroms++;
}
fraim++;
}
return palindroms;
}
}<file_sep>public class Solution {
public string StringShift(string s, int[][] shift) {
var leftShifts = 0;
var rightShifts = 0;
foreach(var sh in shift){
if(sh[0] == 0)
leftShifts+=sh[1];
else
rightShifts+=sh[1];
}
if(rightShifts == leftShifts){
return s;
}
var chars = string.Concat(s,s).ToCharArray();
var totalShifts = 0;
if(rightShifts > leftShifts){
totalShifts = (rightShifts - leftShifts)%s.Length;
return new String(chars, chars.Length/2-totalShifts, s.Length);
}
totalShifts = (leftShifts - rightShifts)%s.Length;
return new String(chars, totalShifts, s.Length);
}
}<file_sep>public class Solution {
public int Calculate(string s) {
var n = s.Length;
var nums = new Stack<int>();
var ops = new Stack<char>();
var precedence = new Dictionary<char, int>{
{'+', 0},
{'-', 0},
{'*', 1},
{'/', 1},
{'(', -1}
};
void calc(){
switch(ops.Pop()){
case '*': nums.Push(nums.Pop()*nums.Pop()); break;
case '/': nums.Push((int)(1.0/nums.Pop()*nums.Pop())); break;
case '+': nums.Push(nums.Pop()+nums.Pop()); break;
case '-': nums.Push(-1*nums.Pop()+nums.Pop()); break;
}
}
for(int i = 0; i < n; i++){
var c = s[i];
if(c >= '0' && c <= '9'){
var num = c - '0';
while(i+1 < n && s[i+1] >= '0' && s[i+1] <= '9'){
num *= 10;
num += s[i+1] - '0';
i++;
}
//Console.WriteLine(num);
nums.Push(num);
} else if(c == '('){
ops.Push(c);
} else if(c == ')'){
while(ops.Peek() != '(')
calc();
ops.Pop();
} else if (c != ' '){
while(ops.Any() && precedence[ops.Peek()] >= precedence[c])
calc();
ops.Push(c);
}
}
while(ops.Any())
calc();
return nums.Pop();
}
}<file_sep>/**
* Definition for singly-linked list.
* public class ListNode {
* public int val;
* public ListNode next;
* public ListNode(int val=0, ListNode next=null) {
* this.val = val;
* this.next = next;
* }
* }
*/
public class Solution {
public ListNode PlusOne(ListNode head) {
var dummy = new ListNode(0, head);
var nonNine = dummy;
while(head != null){
if(head.val < 9)
nonNine = head;
head = head.next;
}
nonNine.val++;
while(nonNine.next != null){
nonNine.next.val = 0;
nonNine = nonNine.next;
}
return dummy.val > 0 ? dummy : dummy.next;
//var carry = PlusOneInternal(head);
//return carry > 0 ? new ListNode(carry, head) : head;
}
public int PlusOneInternal(ListNode node) {
var carry = node.next == null ? 1 : PlusOneInternal(node.next);
var d = node.val + carry;
node.val = d % 10;
return d / 10;
}
}<file_sep>public class Solution {
public bool IsRobotBounded(string instructions) {
var x = 0;
var y = 0;
var d = 0;
var dirs = new []{(0, 1),(1,0),(0,-1),(-1,0)};
foreach(var c in instructions){
switch(c){
case 'R':
d = (d+1) % 4;
break;
case 'L':
d = (d+3) % 4;
break;
case 'G':
var (xd, yd) = dirs[d];
x += xd;
y += yd;
break;
}
}
return (x == 0 && y == 0) || d != 0;
}
}<file_sep>public class Solution {
public ListNode AddTwoNumbers(ListNode l1, ListNode l2) {
int calcLen(ListNode l){
var len = 0;
while(l != null){
len++;
l = l.next;
}
return len;
}
var len1 = calcLen(l1);
var len2 = calcLen(l2);
var res = new ListNode();
int add(ListNode head, int dif, ListNode l1, ListNode l2){
if(l1 == null)
return 0;
var sum = l1.val;
head.next = new ListNode();
if(dif <= 0){
sum += l2.val;
l2 = l2.next;
}
sum += add(head.next, --dif, l1.next, l2);
head.next.val = sum%10;
return sum/10;
}
var dif = Math.Abs(len1-len2);
res.val = len1 > len2 ? add(res, dif, l1, l2) : add(res, dif, l2,l1);
return res.val == 0 ? res.next : res;
}
}<file_sep> public class Solution
{
public int MinPathSum(int[][] grid)
{
if (grid.Length == 0)
return 0;
var h = grid.Length;
var w = grid[0].Length;
var dp = new int[h][];
for(var i = 0; i < h; i++)
{
dp[i] = new int[w];
for(var j = 0; j < w; j++)
{
dp[i][j] = grid[i][j];
if (i>0 && j>0){
dp[i][j] += Math.Min(dp[i-1][j],dp[i][j-1]);
} else if(i > 0){
dp[i][j] += dp[i-1][j];
} else if(j > 0){
dp[i][j] += dp[i][j-1];
}
}
}
return dp[h-1][w-1];
}
}
<file_sep>public class TopVotedCandidate {
int[] winners;
int[] times;
int N;
public TopVotedCandidate(int[] persons, int[] times) {
this.times = times;
N = times.Length;
winners = new int[N];
var scores = new Dictionary<int, int>();
var leader = -1;
for(var i = 0; i < N; i++){
var candidate = persons[i];
if(scores.ContainsKey(candidate))
scores[candidate] += 1;
else
scores[candidate] = 1;
if(leader == -1 || scores[candidate] >= scores[leader]){
leader = candidate;
}
winners[i] = leader;
}
}
public int Q(int t) {
var lo = 0;
var hi = N-1;
while(lo < hi){
var mid = lo + (hi-lo)/2;
if(times[mid] > t)
hi = mid;
else
lo = mid+1;
}
return times[hi] > t ? winners[hi-1] : winners[hi];
}
}
/**
* Your TopVotedCandidate object will be instantiated and called as such:
* TopVotedCandidate obj = new TopVotedCandidate(persons, times);
* int param_1 = obj.Q(t);
*/<file_sep>public class Solution {
public int SubarraySum(int[] nums, int k) {
var runingSum = new Dictionary<int,int>();
var sum = 0;
var result = 0;
for(var i = 0; i < nums.Length; i++){
sum += nums[i];
if (sum == k)
result++;
if (runingSum.TryGetValue(sum-k, out var count)){
result += count;
}
if (runingSum.ContainsKey(sum)){
runingSum[sum]++;
} else{
runingSum[sum] = 1;
}
}
return result;
}
}<file_sep>public class Solution {
public int FindMaxConsecutiveOnes(int[] nums) {
var n = nums.Length;
var len = 0;
var flipped = 0;
var res = 0;
for(int i=0; i<n; i++){
flipped = nums[i] == 1 ? flipped+1 : len+1;
len = nums[i] == 1 ? len+1 : 0;
res = Math.Max(res, flipped);
}
return res;
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public IList<int> RightSideView(TreeNode root) {
var res = new List<int>();
if(root == null)
return res;
var q = new Queue<TreeNode>();
q.Enqueue(root);
while(q.Any()){
var n = q.Count;
for(int i = 0; i < n; i++){
var node = q.Dequeue();
if(node.right != null)
q.Enqueue(node.right);
else if(node.left != null)
q.Enqueue(node.left);
if(i == n-1)
res.Add(node.val);
}
}
return res;
}
}<file_sep>/*
// Definition for a Node.
public class Node {
public int val;
public IList<Node> neighbors;
public Node() {
val = 0;
neighbors = new List<Node>();
}
public Node(int _val) {
val = _val;
neighbors = new List<Node>();
}
public Node(int _val, List<Node> _neighbors) {
val = _val;
neighbors = _neighbors;
}
}
*/
public class Solution {
public Node CloneGraph(Node node) {
if(node == null)
return null;
var visited = new Dictionary<Node, Node>();
var q = new Queue<Node>();
void Clone(Node n){
if(n == null || visited.ContainsKey(n))
return;
q.Enqueue(n);
var clone = new Node(n.val);
visited[n] = clone;
}
Clone(node);
while(q.Any()){
var n = q.Dequeue();
var clone = visited[n];
foreach(var nei in n.neighbors){
Clone(nei);
clone.neighbors.Add(visited[nei]);
}
}
return visited[node];
}
}<file_sep>public class Solution {
public int LongestStrChain(string[] words) {
var a = new Dictionary<string, int>();
var len = new HashSet<int>();
var max = 1;
foreach(var w in words.OrderBy(wd => wd.Length)){
if(a.ContainsKey(w))
continue;
var n = w.Length;
len.Add(n);
if(n==1 || !len.Contains(n-1)){
a[w] = 1;
continue;
}
var sb = new StringBuilder(w);
for(int i = 0; i < n; i++){
var t = sb[i];
sb.Remove(i,1);
var p = sb.ToString();
sb.Insert(i, t);
if(a.ContainsKey(p)){
a[w] = a[p] + 1;
max = Math.Max(max, a[w]);
break;
}
}
if(!a.ContainsKey(w))
a[w] = 1;
}
return max;
}
}<file_sep>public class Solution {
int[] nums;
Random r = new Random();
public Solution(int[] nums) {
this.nums = nums;
}
public int Pick(int target) {
var idx = 0;
var count = 0;
for(int i = 0; i < nums.Length; i++){
if(nums[i] == target){
if(r.Next(0, ++count))
idx = i;
}
}
return idx;
}
}
/**
* Your Solution object will be instantiated and called as such:
* Solution obj = new Solution(nums);
* int param_1 = obj.Pick(target);
*/
/*
public class Solution {
Dictionary<int, List<int>> map = new Dictionary<int, List<int>>();
Random r = new Random();
public Solution(int[] nums) {
for(int i = 0; i < nums.Length; i++){
if(!map.ContainsKey(nums[i]))
map[nums[i]] = new List<int>();
map[nums[i]].Add(i);
}
}
public int Pick(int target) {
var list = map[target];
var idx = r.Next(0, list.Count);
return list[idx];
}
}
*/
<file_sep>public class Solution {
public bool CanTransform(string start, string end) {
var n = start.Length;
if (n != end.Length)
return false;
if(start.Count(c => c == 'R') != end.Count(c => c == 'R'))
return false;
if(start.Count(c => c == 'L') != end.Count(c => c == 'L'))
return false;
var s = 0;
var e = 0;
while(s < n){
while(s < n && start[s] == 'X')
s++;
while(e < n && end[e] == 'X')
e++;
if(s == n || e == n)
return e == s;
if(start[s] != end[e] )
return false;
s++;
e++;
}
return true;
}
}<file_sep>public class Solution {
public IList<string> FindMissingRanges(int[] nums, int lower, int upper) {
var res = new List<string>();
var ptr = 0;
if(nums.Length == 0){
res.Add(CreateRange(lower, upper));
return res;
}
while(lower <= upper && ptr < nums.Length){
if(lower == nums[ptr]){
lower++;
ptr++;
} else {
res.Add(CreateRange(lower, nums[ptr]-1));
lower = nums[ptr];
}
}
if (lower <= upper && ptr == nums.Length){
res.Add(CreateRange(nums[nums.Length-1]+1, upper));
}
return res;
}
private string CreateRange(int lo, int hi){
return hi == lo ? hi.ToString() : $"{lo}->{hi}";
}
}<file_sep>public class Solution {
public string ModifyString(string s) {
var sb = s.ToCharArray();
var a = (int)'a';
for(int i = 0; i < sb.Length; i++){
if(sb[i] == '?'){
var c = a;
while(i > 0 && c == sb[i-1] || i < sb.Length-1 && c == sb[i+1])
c++;
sb[i] = (char)c;
}
}
return new string(sb);
}
}<file_sep>public class WordDictionary {
Node trie = new Node();
HashSet<string> set = new HashSet<string>();
public WordDictionary() {
}
public void AddWord(string word) {
var node = trie;
foreach(var c in word){
var idx = c - 'a';
if(node.Children[idx] == null)
node.Children[idx] = new Node();
node = node.Children[idx];
}
node.IsWord = true;
set.Add(word);
}
public bool Search(string word) {
return set.Contains(word) || TryFind(trie, word, 0);
}
public bool TryFind(Node node, string w, int idx){
if(node == null)
return false;
if(idx == w.Length)
return node.IsWord;
if(w[idx] != '.')
return TryFind(node.Children[w[idx]-'a'], w, idx+1);
foreach(var child in node.Children.Where(c => c != null)){
if(TryFind(child, w, idx + 1))
return true;
}
return false;
}
}
public class Node{
public Node[] Children = new Node[26];
public bool IsWord;
}
<file_sep>public class Solution {
public int[] MaxSumOfThreeSubarrays(int[] nums, int k) {
/*var res = DFS(nums, k, 0, 3, new int[nums.Length, 4][]);
return res.Skip(1).ToArray();*/
var sums = new int[nums.Length - k + 1];
var sum = 0;
for(int i = 0; i < nums.Length; i++){
sum += nums[i];
if(i - k >= 0)
sum -= nums[i-k];
// have K in window. start counting
if(i - k + 1 >= 0)
sums[i - k + 1] = sum;
}
var n = sums.Length;
var bestSoFar = new int[n,2];
var best = 0;
for(int i = 0; i < n; i++){
if(sums[i] > sums[best])
best = i;
bestSoFar[i,0] = best;
}
best = n-1;
for(int i = n-1; i >= 0; i--){
if(sums[i] >= sums[best])
best = i;
bestSoFar[i,1] = best;
}
var res = new int[3];
sum = 0;
for(int i = k; i < n - k; i++){
var l = bestSoFar[i-k, 0];
var r = bestSoFar[i+k, 1];
var curSum = sums[l] + sums[i] + sums[r];
if(curSum > sum){
sum = curSum;
res[0] = l;
res[1] = i;
res[2] = r;
}
}
return res;
}
/*public int[] DFS(int[] nums, int k, int start, int left, int[,][] memo){
if(memo[start, left] != null)
return memo[start, left];
var localSum = 0;
var maxLocalSum = int.MinValue;
var maxSum = int.MinValue;
var res = new int[4];
for(int i = start; i < nums.Length-(k*(left-1)); i++){
localSum += nums[i];
if(i - k >= start)
localSum -= nums[i-k];
// have K in window. start counting
if(i-(k-1) >= start){
maxLocalSum = Math.Max(maxLocalSum, localSum);
var sum = maxLocalSum;
if(left > 1){
var dfs = DFS(nums, k, i+1, left-1, memo);
sum += dfs[0];
if(sum > maxSum)
res = dfs;
}
if(sum > maxSum) {
maxSum = sum;
res[0] = maxSum;
res[4-left] = i-(k-1);
}
}
}
memo[start, left] = res;
return res;
}*/
}<file_sep>public class Solution {
public bool IsIsomorphic(string s, string t) {
var map = new Dictionary<char,char>();
var taken = new Dictionary<char,char>();
var n = s.Length;
for(int i = 0; i < n; i++){
var from = s[i];
var to = t[i];
if(map.ContainsKey(from) && to != map[from])
return false;
if(taken.ContainsKey(to) && from != taken[to])
return false;
map[from] = to;
taken[to] = from;
}
return true;
}
}<file_sep>public class Solution {
public int ConfusingNumberII(int N) {
var map = new long[]{0,1,6,8,9};
var count = 0;
void Calc(long curr){
if(curr > 0){
var rotated = 0L;
var orig = curr;
while(orig > 0){
var last = orig%10;
orig /= 10;
rotated *= 10;
rotated += last == 9 ? 6 : last == 6 ? 9 : last;
}
if(rotated != curr)
count++;
}
foreach(var kv in map){
var num = curr*10+kv;
if(num <= N && num > 0)
Calc(num);
}
}
Calc(0);
return count;
}
}<file_sep>public class Solution {
public int MaximumSwap(int num) {
var nums = num.ToString().ToCharArray();
var lastPos = new int[10];
for(int i = 0; i < nums.Length; i++)
lastPos[nums[i]-'0'] = i;
for(int i = 0; i < nums.Length; i++){
for(var n = 9; n > nums[i]-'0'; n--){
if(lastPos[n] > i){
var t = nums[i];
nums[i] = nums[lastPos[n]];
nums[lastPos[n]] = t;
return int.Parse(new string(nums));
}
}
}
return num;
}
}<file_sep>/**
* Definition for singly-linked list.
* public class ListNode {
* public int val;
* public ListNode next;
* public ListNode(int x) { val = x; }
* }
*/
public class Solution {
public ListNode RemoveNthFromEnd(ListNode head, int n) {
if (head == null || head.next == null)
return null;
ListNode start = new ListNode(0);
start.next = head;
var ahead = start;
var behind = start;
while(n-- >= 0)
{
ahead = ahead.next;
}
while(ahead != null){
ahead = ahead.next;
behind = behind.next;
}
behind.next = behind.next.next;
return start.next;
}
}<file_sep>public class Solution {
public int[] Intersection(int[] nums1, int[] nums2) {
if(nums1.Length > nums2.Length)
return Intersection(nums2, nums1);
var set = new HashSet<int>(nums2);
var res = new HashSet<int>();
foreach(var n in nums1)
if(set.Contains(n))
res.Add(n);
return res.ToArray();
}
}<file_sep>/*
// Definition for a Node.
public class Node {
public int val;
public Node left;
public Node right;
public Node next;
public Node() {}
public Node(int _val) {
val = _val;
}
public Node(int _val, Node _left, Node _right, Node _next) {
val = _val;
left = _left;
right = _right;
next = _next;
}
}
*/
public class Solution {
public Node Connect(Node root) {
void link(Node next, ref Node cur, ref Node first){
if(first == null)
first = cur ?? next;
if(cur == null){
cur = next;
}else if(next != null){
cur.next = next;
cur = next;
}
}
var left = root;
while(left != null){
var head = left;
var cur = head.left;
left = null;
while(head != null){
link(head.right, ref cur, ref left);
if(head.next != null)
link(head.next.left, ref cur, ref left);
head = head.next;
}
}
return root;
}
}<file_sep>public class Solution {
public IList<int> PartitionLabels(string s) {
var n = s.Length;
var lastIdx = new int[26];
for(int i = 0; i < n ; i++){
lastIdx[s[i] - 'a'] = i;
}
var res = new List<int>();
var start = -1;
var end = 0;
for(int i = 0; i < n; i++){
end = Math.Max(end, lastIdx[s[i]-'a']);
if(i == end){
res.Add(end-start);
start = end;
}
}
return res;
}
}<file_sep>public class Solution {
public string NumberToWords(int num) {
if(num == 0)
return "Zero";
var ones = new string[] {"One","Two","Three","Four","Five","Six","Seven","Eight", "Nine", "Ten", "Eleven", "Twelve", "Thirteen", "Fourteen", "Fifteen", "Sixteen", "Seventeen", "Eighteen", "Nineteen"};
var tens = new string[] {"Twenty", "Thirty", "Forty", "Fifty", "Sixty", "Seventy", "Eighty", "Ninety"};
IEnumerable<string> ReadTens(){
var n1 = num % 10;
var n2 = num % 100;
if(n2 == 0)
yield break;
else if(n2 < 20)
yield return ones[n2-1];
else{
if(n1 != 0)
yield return ones[n1-1];
yield return tens[n2/10-2];
}
}
var exp = new string[] {"Thousand", "Million", "Billion"};
var count = 0;
var res = new Stack<string>();
while(num > 0){
if(num % 1000 == 0){
count++;
num /= 1000;
continue;
}
if(count > 0)
res.Push(exp[count-1]);
count++;
foreach(var str in ReadTens())
res.Push(str);
num /= 100;
var n = num % 10;
if(n > 0){
res.Push("Hundred");
res.Push(ones[n-1]);
}
num /= 10;
}
return string.Join(" ", res);
}
}<file_sep>public class Solution {
public int MaxProfit(int[] prices) {
int total = 0;
for (int i=0; i< prices.Length-1; i++) {
if (prices[i+1]>prices[i]) total += prices[i+1]-prices[i];
}
return total;
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public IList<TreeNode> DelNodes(TreeNode root, int[] to_delete) {
var res = new List<TreeNode>();
var d = new HashSet<int>(to_delete);
DeleteNodes(root, res, d, null, false);
if(!d.Contains(root.val))
res.Add(root);
return res;
}
private void DeleteNodes(TreeNode n, List<TreeNode> res, HashSet<int> d, TreeNode parent, bool left){
if(n == null)
return;
if(d.Contains(n.val)){
if(parent != null){
if(left)
parent.left = null;
else
parent.right = null;
}
if(n.left != null && !d.Contains(n.left.val))
res.Add(n.left);
if(n.right != null && !d.Contains(n.right.val))
res.Add(n.right);
}
DeleteNodes(n.left, res, d, n, true);
DeleteNodes(n.right, res, d, n, false);
}
}<file_sep>public class Solution {
public int RangeSumBST(TreeNode root, int low, int high) {
if(root == null)
return 0;
if(root.val < low)
return RangeSumBST(root.right, low, high);
if(root.val > high)
return RangeSumBST(root.left, low, high);
return root.val + RangeSumBST(root.right, low, high) + RangeSumBST(root.left, low, high);
}
}<file_sep>public class Solution {
public string AddStrings(string num1, string num2) {
var l1 = num1.Length;
var l2 = num2.Length;
if(l1 < l2)
return AddStrings(num2, num1);
var res = new Stack<char>();
var remainder = 0;
for(int i = l1-1; i >= 0; i--){
var n1 = num1[i]-'0';
var idx = i + l2 - l1;
var n2 = idx >= 0 ? num2[idx]-'0' : 0;
var sum = n1+n2+remainder;
remainder = sum/10;
sum %= 10;
res.Push((char)(sum + '0'));
}
if(remainder > 0)
res.Push((char)(remainder+'0'));
return new String(res.ToArray());
}
}<file_sep>public class Solution {
public int MinimumTotal(IList<IList<int>> triangle) {
var res = 0;
var rows = triangle.Count;
for(int r = rows-2; r >= 0; r--){
for(int c = 0; c < triangle[r].Count; c++){
triangle[r][c] += Math.Min(triangle[r+1][c], triangle[r+1][c+1]);
}
}
return triangle[0][0];
}
}<file_sep>public class Solution {
public string MinWindow(string S, string T) {
if(S == T)
return S;
// optimization
if(T.Length == 1){
for (int i = 0; i < S.Length; i++)
{
if(S[i] == T[0])
return T;
}
return "";
}
int c = 0;
int start = -1;
int len = -1;
for (int i = 0; i < S.Length; i++)
{
// go from left to right , to find the end index
if (S[i] == T[c])
{
c++;
}
// found a match
if (c == T.Length)
{
//go from right to left , to find the start index
int d = i;
while(c > 0)
{
if (T[c - 1] == S[d])
{
c--;
}
d--;
}
if (len == -1 || i - d < len)
{
len = i - d;
start = d + 1;
}
//reset the new search index to the old start position
i = d + 1;
}
}
if (len == -1) return "";
return S.Substring(start , len);
}
}<file_sep>public class Solution {
public bool ValidMountainArray(int[] arr) {
var n = arr.Length;
if(n<3)
return false;
var down = false;
for(var i=0; i < n-1; i++){
var cur = arr[i];
var next = arr[i+1];
if(next > cur){
if(down)
return false;
} else if(next < cur){
if(!down){
if(i==0)
return false;
down = true;
}
} else{
return false;
}
}
return down;
}
}<file_sep>public class Solution {
public int[] FindRedundantConnection(int[][] edges) {
int[] res = null;
var dsu = new DSU(edges.Length);
foreach(var edge in edges){
if(dsu.Union(edge[0], edge[1]))
res = edge;
}
return res;
}
class DSU{
int[] arr;
public DSU(int n){
arr = Enumerable<int>.Range(0, n).ToArray();
}
public bool Union(int a, int b){
var parenta = Find(a);
var parentb = Find(b);
arr[parenta] = parentb;
return parenta == parentb;
}
public int Find(int a){
if(arr[a] != a)
arr[a] = Find(arr[a]); // path compression
return arr[a];
}
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public TreeNode SortedArrayToBST(int[] nums) {
return Convert(nums, 0, nums.Length-1);
}
public TreeNode Convert(int[] nums, int start, int end){// 0 1 // 3 4
if(end < start)
return null;
if(start == end) // f
return new TreeNode(nums[start]);
var mid = start + (end-start)/2; // 3
var node = new TreeNode(nums[mid]); // 5
node.Left = Convert(nums, start, mid-1); // 3 2
node.Right = Convert(nums, mid+1, end); // 4 4
return node; // 0
}
}<file_sep>public class Solution {
public bool IsMatch(string s, string p) {
//return DFS(s, p, 0, 0, new int?[s.Length+1, p.Length+1]);
var dp = new int[s.Length+1, p.Length+1];
dp[s.Length,p.Length] = true;
for (int posS = s.Length; posS >= 0; posS--){
for (int posP = p.Length - 1; posP >= 0; posP--){
var symbolMatch = posS < s.Length && (s[posS] == p[posP] || p[posP] == '.');
var isAsterisk = posP < p.Length-1 && p[posP+1] == '*';
var res = false;
if(isAsterisk){
// skip * and move on OR continue with current *
dp[posS, posP] = dp[posS, posP+2] || symbolMatch && dp[posS+1, posP];
} else {
dp[posS, posP] = symbolMatch && dp[posS+1, posP+1]; // simple case
}
}
}
return dp[0,0];
}
public bool DFS(string s, string p, int posS, int posP, int?[,] memo) {
if(posP == p.Length)
return posS == s.Length;
if(memo[posS, posP] != null)
return memo[posS, posP].Value;
var symbolMatch = posS < s.Length && (s[posS] == p[posP] || p[posP] == '.');
var isAsterisk = posP < p.Length-1 && p[posP+1] == '*';
var res = false;
if(isAsterisk){
res = DFS(s, p, posS, posP+2); // skip * and move on
res = res || symbolMatch && DFS(s, p, posS+1, posP); // continue with current *
} else {
res = symbolMatch && DFS(s, p, posS+1, posP+1); // simple case
}
memo[posS, posP] = res;
return res;
}
}
/*
"c"
"c*f"
*/<file_sep>public class Solution {
public int MinCost(string s, int[] cost) {
var n = cost.Length;
var sum = cost[0];
var max = cost[0];
for(int i = 1; i < n; i++){
if(s[i] != s[i-1]){ // when repetitive seq is ended remove max from sum
sum -= max;
max = 0;
}
// calc sum and max for repeating
sum += cost[i];
max = Math.Max(max, cost[i]);
}
return sum-max;
}
}<file_sep>public class Solution {
public int[] FindOrder(int numCourses, int[][] prerequisites) {
var g = new List<int>[numCourses];
var indegree = new int[numCourses];
foreach(var p in prerequisites){
var key = p[1];
if(g[key] == null)
g[key] = new List<int>();
g[key].Add(p[0]);
indegree[p[0]]++;
}
var q = new Queue<int>();
var res = new int[numCourses];
for(var i = 0; i < indegree.Length; i++)
if(indegree[i] == 0){
q.Enqueue(i);
res.Add(i);
}
while(q.Any()){
var course = q.Dequeue();
var children = g[course];
if(children == null)
continue;
foreach(var child in children){
indegree[child]--;
if(indegree[child] == 0){
q.Enqueue(child);
res.Add(child);
}
}
}
return res;
}
}
public class Solution {
private HashSet<int> visited = new HashSet<int>();
private Stack<int> order = new Stack<int>();
private HashSet<int>[] g;
public int[] FindOrder(int numCourses, int[][] prerequisites) {
g = new HashSet<int>[numCourses];
foreach(var p in prerequisites){
var key = p[1];
if(g[key] == null)
g[key] = new HashSet<int>();
g[key].Add(p[0]);
}
for(int i = 0; i < numCourses; i++){
if (!BFS(i, new HashSet<int>()))
return new int[0];
}
return order.ToArray();
}
private bool BFS(int node, HashSet<int> visiting){
if(visited.Contains(node))
return true;
if(visiting.Contains(node))
return false;
visiting.Add(node);
if(g[node] != null)
foreach(var child in g[node]){
if (!BFS(child, visiting))
return false;
}
visited.Add(node);
order.Push(node);
return true;
}
}<file_sep>public class Solution {
public int[] ProductExceptSelf(int[] nums) {
var n = nums.Length;
var res = new int[n];
res[0] = nums[0];
for(int i = 1; i < n; i++){
res[i] = res[i-1] * nums[i];
}
var product = 1;
for(int i = n - 2; i >= 0; i--){
res[i+1] = res[i]*product;
product *= nums[i+1];
}
res[0] = product;
return res;
}
}<file_sep>public class Solution {
public int FindDuplicate(int[] nums) {
var n = nums.Length;
for(int i = 0 ; i < n; i++){
var idx = Math.Abs(nums[i])-1;
if(nums[idx] < 0)
return idx+1;
nums[idx] *= -1;
}
return -1;
}
}<file_sep>public class Solution {
public bool SequenceReconstruction(int[] org, IList<IList<int>> seqs) {
var n = org.Length;
var order = new int[n+1];
var pairs = new bool[n];
for(var i =0; i < n; i++){
order[org[i]] = i;
}
foreach(var seq in seqs){
for(var i = 0; i< seq.Count; i++){
if (seq[i] <= 0 || seq[i]> n) return false;
if (i == 0 && seq[i] == org[0]) pairs[0] = true;
if (i > 0 && order[seq[i-1]] >= order[seq[i]]) return false;
if (i > 0 && order[seq[i-1]] + 1 == order[seq[i]]) pairs[order[seq[i]]] = true;
}
}
for (int i = 0; i < n; i++) {
if (!pairs[i]) return false;
}
return true;
}
}<file_sep>public class Solution {
public int[][] IntervalIntersection(int[][] firstList, int[][] secondList) {
var idx1 = 0;
var idx2 = 0;
var res = new List<int[]>();
while(idx1 < firstList.Length && idx2 < secondList.Length){
var start1 = firstList[idx1][0];
var start2 = secondList[idx2][0];
var end1 = firstList[idx1][1];
var end2 = secondList[idx2][1];
if(start2 >= start1 && start2 <= end1 || start1 >= start2 && start1 <= end2)
res.Add(new int[]{Math.Max(start1, start2), Math.Min(end1, end2)});
if(end1 <= end2)
idx1++;
if(end2 <= end1)
idx2++;
}
return res.ToArray();
}
}<file_sep>public class Solution {
public int LengthOfLongestSubstring(string s) {
var n = s.Length;
var map = new int[128];
int l = 0, r = 0, res = 0;
while(r < n){
var c = s[r];
l = Math.Max(map[c], l);
res = Math.Max(res, r-l+1);
map[c] = ++r;
}
return res;
}
}<file_sep>public class Solution {
public int CheckRecord(int n) {
var M = (long)Math.Pow(10, 9)+7;
var dp = new long[n<=5 ? 6 : n+1];
dp[0] = 1;
dp[1] = 2;
dp[2] = 4;
dp[3] = 7; // 8-1
for(var i = 4; i <= n; i++){
dp[i] = ((2*dp[i-1])%M + (M - dp[i-4])) % M;
}
var sum = dp[n];
for(var i = 1; i <= n; i++){
sum += (dp[i-1] * dp[n-i]) % M;
}
return (int)(sum % M);
}
}<file_sep>public class Solution {
public string CountAndSay(int n) { // 4
var num = "1";
for(; n > 1; n--){ //true 1
var count = 1;
var prev = num[0]; // 1
var sb = new StringBuilder();
for(int i = 1; i < num.Length; i++){ // true
var cur = num[i]; // 1
if(cur != prev){
sb.Append(count.ToString()); // 111
sb.Append(prev); // 1112
count = 1;
prev = cur; // 1
} else {
count++; // 2
}
}
sb.Append(count.ToString()); // 11122
sb.Append(prev); // 111221
num = sb.ToString(); // 1211
}
return num;
}
}<file_sep>public class Solution {
public int[][] Merge(int[][] intervals) {
var n = intervals.Length;
if(n <= 1)
return intervals;
Array.Sort(intervals, new IntervalComparer());
var res = new List<int[]>();
var cur = intervals[0];
for(int i = 1; i < n; i++){
if(cur[1] >= intervals[i][0]){
cur[0] = Math.Min(cur[0], intervals[i][0]);
cur[1] = Math.Max(cur[1], intervals[i][1]);
} else {
res.Add(cur);
cur = intervals[i];
}
}
res.Add(cur);
return res.ToArray();
}
public class IntervalComparer : IComparer<int[]>{
public int Compare(int[] a, int[] b){
return a[0] - b[0];
}
}
}<file_sep>public class Solution {
public int MaxArea(int[] height) {
int l = 0, res = 0;
var r = height.Length-1;
while(l < r){
res = Math.Max(res, Math.Min(height[l], height[r])*(r-l));
if (height[l] > height[r])
r--;
else
l++;
}
return res;
}
}<file_sep>public class Solution {
public string GetHint(string secret, string guess) {
var bulls = 0;
var cows = 0;
var n = secret.Length;
if(n == 0)
return $"{bulls}A{cows}B";
var map = new Dictionary<char, int>();
foreach(var c in secret){
if(map.ContainsKey(c))
map[c] += 1;
else
map[c] = 1;
}
for(var i = 0; i < n; i++){
var c = guess[i];
if(map.ContainsKey(c)){
if(secret[i] == c){
bulls++;
if(map[c] <= 0)
cows--;
} else {
if(map[c] > 0)
cows++;
}
map[c] -= 1;
}
}
return $"{bulls}A{cows}B";
}
}<file_sep>using System.CodeDom.Compiler;
using System.Collections.Generic;
using System.Collections;
using System.ComponentModel;
using System.Diagnostics.CodeAnalysis;
using System.Globalization;
using System.IO;
using System.Linq;
using System.Reflection;
using System.Runtime.Serialization;
using System.Text.RegularExpressions;
using System.Text;
using System;
class Solution {
// Complete the activityNotifications function below.
static int activityNotifications(int[] ex, int d) {
var arr = new int[200];
var i = 0;
for(; i < d; i++){
arr[ex[i]]++;
}
var mid = (d+1)/2;
var res = 0;
i--;
for(; i < ex.Length-1; i++){
var counter = 0;
var median = 0.0;
for(int j = 0; j < arr.Length; j++){
counter += arr[j];
if(counter >= mid){
if(d%2 != 0){
median = j;
} else {
var k = j;
while(counter == mid){
counter += arr[++k];
}
//Console.WriteLine(j + " " + k);
median = (j + k) / 2.0;
}
break;
}
}
//Console.WriteLine(median);
if(ex[i+1] >= median*2)
res++;
var drop = ex[i-(d-1)];
arr[drop]--;
arr[ex[i+1]]++;
}
return res;
}
static void Main(string[] args) {
TextWriter textWriter = new StreamWriter(@System.Environment.GetEnvironmentVariable("OUTPUT_PATH"), true);
string[] nd = Console.ReadLine().Split(' ');
int n = Convert.ToInt32(nd[0]);
int d = Convert.ToInt32(nd[1]);
int[] expenditure = Array.ConvertAll(Console.ReadLine().Split(' '), expenditureTemp => Convert.ToInt32(expenditureTemp));
int result = activityNotifications(expenditure, d);
textWriter.WriteLine(result);
textWriter.Flush();
textWriter.Close();
}
}
<file_sep>/**
* Definition for singly-linked list.
* public class ListNode {
* public int val;
* public ListNode next;
* public ListNode(int val=0, ListNode next=null) {
* this.val = val;
* this.next = next;
* }
* }
*/
public class Solution {
public ListNode ReverseList(ListNode head) {
return ReverseList(head, null);
}
public ListNode ReverseList(ListNode head, ListNode prev) {
if(head == null)
return prev;
var next = head.next;
head.next = prev;
return ReverseList(next, head);
}
/*public ListNode ReverseList(ListNode head) {
ListNode prev = null;
var curr = head;
while(curr != null){
var next = curr.next;
curr.next = prev;
prev = curr;
curr = next;
}
return prev;
}*/
}<file_sep>public class Solution {
public string RemoveDuplicates(string s, int k) {
if(k == 1)
return "";
var st = new LinkedList<Symbol>();
foreach(var c in s){
var peek = st?.Last?.Value;
if(peek == null || peek.Char != c){
st.AddLast(new Symbol{ Char = c, Count = 1});
} else if(++peek.Count == k){
st.RemoveLast();
}
}
var sb = new StringBuilder();
foreach(var symbol in st){
while(symbol.Count-- > 0)
sb.Append(symbol.Char);
}
return sb.ToString();
}
private class Symbol{
public char Char;
public int Count;
}
}<file_sep>public class Solution {
public IList<IList<string>> GroupAnagrams(string[] strs) {
var buckets = new Dictionary<string, List<string>>();
foreach (var str in strs)
{
var charArray = str.ToCharArray();
Array.Sort(charArray);
var sortedStr = new string(charArray);
if (!buckets.TryGetValue(sortedStr, out var val))
{
buckets[sortedStr] = new List<string>{str};
}
else
{
val.Add(str);
}
}
IList<IList<string>> matrix = new List<IList<string>>();
foreach (var bucket in buckets.Values)
{
matrix.Add(bucket);
}
return matrix;
}
}<file_sep>public class Solution {
public int CompareVersion(string version1, string version2) {
int i = 0, j = 0;
int currentVersion1, currentVersion2;
while(i < version1.Length || j < version2.Length) {
currentVersion1 = 0;
while(i < version1.Length && version1[i] != '.'){
currentVersion1 = currentVersion1 * 10 + (version1[i] - '0');
++i;
}
currentVersion2 = 0;
while(j < version2.Length && version2[j] != '.'){
currentVersion2 = currentVersion2 * 10 + (version2[j] - '0');
++j;
}
if(currentVersion1 > currentVersion2)
return 1;
if(currentVersion2 > currentVersion1)
return -1;
++i;
++j;
}
return 0;
}
}<file_sep>/*
You're given an array of positive integers representing the prices of a single stock on
various days (each index in the array represents a different day). You're also
given an integer k, which represents the number of transactions
you're allowed to make. One transaction consists of buying the stock on a
given day and selling it on another, later day.
Write a function that returns the maximum profit that you can make by buying
and selling the stock, given k transactions.
Note that you can only hold one share of the stock at a time; in other words,
you can't buy more than one share of the stock on any given day, and you can't
buy a share of the stock if you're still holding another share. Also, you
don't need to use all k transactions that you're allowed.
Input = [5, 11, 3, 50, 60, 90]
k = 2
Output = 93
5, 11, 3, 50, 60, 90
3, 5
60, 90
day0 0, 6, -2, 45, 55, 85
day1 0, -8, 39, 49, 79
55 11 3 60 90
*/
class Program {
public static int maxProfitWithKTransactions(int[] prices, int k) {
if(prices == null || prices.Length < 2*k) // false
return -1;
return DFS(prices, 0, k, new int?[prices.Length, k]); // [1,2], 0, 1, new[][]
}
int DFS(int[] prices, int start, int k, int?[,] memo){ //
if(memo[start, k] != null) //
return memo[start,k];//
var maxProfitTotal = int.MinValue;//
for(int i = start; i <= prices.Length-(k-1)*2; i++){//
var profit = prices[start] - prices[i];
if(k > 1)
profit += DFS(prices, i+1, k-1, memo);
maxProfitTotal = Math.Max(maxProfitTotal, profit);//
}
memo[start, k] = maxProfitTotal;//
return maxProfitTotal;
}
}
/*
class Program {
// O(nk) time | O(nk) space
public static int maxProfitWithKTra if (prices.length == 0) { return 0; }
int[][] profits = new int[k + 1]
for (int t = 1; t < k + 1; t++) {
int maxThusFar = Integer.MIN_VAL;
for (int d = 1; d < prices.length; d++) {
maxThusFar = Math.max(maxThus profits[t][d] = Math.max(profits[t-1][d-1] - prices[d - 1];
profits[t][d] = Math.max(profits[t][d-1], maxThusFar - prices[d]);
return profits[k][prices.length - 1];
}
}
}
*/
<file_sep>public class SnapshotArray {
List<ValueTuple<int,int>>[] data;
int snapshots;
public SnapshotArray(int length) {
data = new List<ValueTuple<int,int>>[length];
for(int i = 0; i < length; i++){
data[i] = new List<ValueTuple<int,int>>{(0, 0)};
}
}
public void Set(int index, int val) {
var versions = data[index];
(var version, _) = versions[versions.Count-1];
if(snapshots > version){
versions.Add((snapshots, val));
} else {
versions[versions.Count-1] = (version, val);
}
}
public int Snap() {
return snapshots++;
}
public int Get(int index, int snap_id) {
var versions = data[index];
var lo = 0;
var hi = versions.Count;
while(lo < hi){
var mid = lo + (hi-lo)/2;
(var version, var val) = versions[mid];
if (version == snap_id)
return val;
if(version > snap_id)
hi = mid;
else
lo = mid + 1;
}
(var ver, var v) = versions[hi-1];
return v;
}
}
/**
* Your SnapshotArray object will be instantiated and called as such:
* SnapshotArray obj = new SnapshotArray(length);
* obj.Set(index,val);
* int param_2 = obj.Snap();
* int param_3 = obj.Get(index,snap_id);
*/<file_sep>public class Solution {
private ValueTuple<int, int>[] dir = new ValueTuple<int, int>[]{
(-1,0),
(-1,1),
(-1,-1),
(0,-1),
(1,1),
(0,1),
(1,0),
(1,-1)
};
public int ShortestPathBinaryMatrix(int[][] grid) {
var rows = grid.Length;
if(rows == 0)
return -1;
var cols = grid[0].Length;
if(grid[0][0] == 1 || grid[rows-1][cols-1] == 1)
return -1;
var q = new Queue<ValueTuple<int,int,int>>();
q.Enqueue((0,0,0));
grid[0][0] = 1;
while(q.Any()){
(var rowS, var colS, var path) = q.Dequeue();
if(rowS == rows - 1 && colS == cols - 1)
return path+1;
foreach((var rowD, var colD) in dir){
var row = rowS + rowD;
var col = colS + colD;
if(row >= 0 && col >= 0 && row < rows && col < cols && grid[row][col] != 1){
q.Enqueue((row,col, path+1));
grid[row][col] = 1;
}
}
}
return -1;
}
}<file_sep>public class Solution {
public int MinDistance(string word1, string word2) {
if (word1 == null || word2 == null)
return -1;
var n = word1.Length;
var m = word2.Length;
if (n == 0)
return m;
if (m == 0)
return n;
var dp = new int[n+1, m+1];
for(int i = 0; i <= n; i++)
dp[i, 0] = i;
for(int j = 0; j <= m; j++)
dp[0, j] = j;
for(int i = 0; i < n; i++)
for(int j = 0; j < m; j++)
dp[i+1, j+1] = word1[i] == word2[j]
? dp[i, j]
: Math.Min(dp[i+1, j], Math.Min(dp[i, j+1], dp[i, j])) + 1;
return dp[n, m];
}
}
public class Solution {
public int MinDistance(string word1, string word2) {
if (word1 == null || word1.Length == 0)
return word2.Length;
if (word2 == null || word2.Length == 0)
return word1.Length;
return dfs(word1, word2, 0, 0, new int?[word1.Length+1, word2.Length+1]);
}
int dfs(string s1, string s2, int i, int j, int?[,] memo){
if(i >= s1.Length)
return s2.Length-j;
if(j >= s2.Length)
return s1.Length-i;
if(memo[i, j] != null)
return memo[i,j].Value;
var len = 0;
if(s1[i] == s2[j]){
len = dfs(s1, s2, i+1, j+1, memo);
} else {
var ins = dfs(s1, s2, i+1, j, memo);
var del = dfs(s1, s2, i, j+1, memo);
var repl = dfs(s1, s2, i+1, j+1, memo);
len = Math.Min(ins, Math.Min(del, repl)) + 1;
}
memo[i,j] = len;
return len;
}
}<file_sep>using System;
// you can also use other imports, for example:
// using System.Collections.Generic;
// you can write to stdout for debugging purposes, e.g.
// Console.WriteLine("this is a debug message");
class Solution {
public int solution(int N) {
var max = 0;
var cur = 0;
var startCount = false;
for(int i = 30; i >=0; i--){
var exponent = 1 << i;
if(N >= exponent){
max = Math.Max(max, cur);
N -= exponent;
cur = 0;
startCount = true;
} else if(startCount){
cur++;
}
}
return max;
}
}<file_sep>public class Solution {
public int[] SortArray(int[] nums) {
//MergeSort(nums, new int[nums.Length], 0, nums.Length-1);
QuickSort(nums, 0, nums.Length-1);
return nums;
}
public void MergeSort(int[] nums, int[] helper, int lo, int hi) {
if(lo < hi){
var mid = lo + (hi-lo)/2;
MergeSort(nums, helper, lo, mid);
MergeSort(nums, helper, mid+1, hi);
Merge(nums, helper, lo, hi, mid);
}
}
public void Merge(int[] nums, int[] helper, int lo, int hi, int mid) {
for(int i = lo; i<= hi; i++)
helper[i] = nums[i];
var loh = lo;
var hih = mid+1;
while(loh <= mid && hih <= hi){
if(helper[loh] <= helper[hih])
nums[lo++] = helper[loh++];
else
nums[lo++] = helper[hih++];
}
while(loh <= mid)
nums[lo++] = helper[loh++];
}
/////////////////////////////////////////////////////
public void QuickSort(int[] nums, int lo, int hi) {
if(lo < hi){
var mid = Partition(nums, lo, hi);
QuickSort(nums, lo, mid - 1);
QuickSort(nums, mid + 1, hi);
}
}
public int Partition(int[] nums, int lo, int hi) {
var pivot = nums[hi];
for(int i = lo; i < hi; i++){
if(nums[i] < pivot)
Swap(nums, lo++, i);
}
Swap(nums, lo, hi);
return lo;
}
public void Swap(int[] nums, int a, int b){
var t = nums[a];
nums[a] = nums[b];
nums[b] = t;
}
}<file_sep>public class Solution {
private int cols;
private int rows;
private int[][] dir = {new []{0,1}, new []{1,0}, new []{0,-1}, new []{-1,0}};
private int[,] memo;
public int LongestIncreasingPath(int[][] matrix) {
rows = matrix.Length;
if(rows == 0)
return 0;
cols = matrix[0].Length;
memo = new int[rows, cols];
var max = 0;
for(int i = 0; i < rows; i++)
for(int j = 0; j < cols; j++)
max = Math.Max(max, DFS(matrix, i, j));
return max;
}
private int DFS(int[][] m, int row, int col){
if(memo[row, col] > 0)
return memo[row, col];
var len = 0;
foreach(var d in dir){
var r = row+d[0];
var c = col+d[1];
if(r >= 0 && r < rows && c >= 0 && c < cols && m[row][col] < m[r][c])
len = Math.Max(len, DFS(m, r, c));
}
memo[row, col] = ++len;
return len;
}
}<file_sep>public class Solution {
public int KthGrammar(int N, int K) {
if(N == 1)
return 0;
var prev = (K+1)/2.0;
var prevK = (int)prev;
var takeLeft = prev == (double)prevK;
var parent = KthGrammar(N-1, prevK);
return takeLeft ? parent : (parent+1)%2;
}
}<file_sep>public class Solution {
public int StoneGameII(int[] piles) {
var n = piles.Length;
var maxScore = new int[n];
maxScore[n-1] = piles[n-1];
for(int i = n-2; i >= 0; i--)
maxScore[i] = maxScore[i+1] + piles[i];
var memo = new Dictionary<(int, int), int>();
int DFS(int pile, int m){
if(pile >= n)
return 0;
if(n-pile <= 2*m)
return maxScore[pile];
var key = (pile, m);
if(memo.ContainsKey(key))
return memo[key];
var min = int.MaxValue;
for(var i=1; i <= 2*m; i++){
min = Math.Min(min, DFS(pile+i, Math.Max(i,m)));
}
memo[key] = maxScore[pile] - min;
return memo[key];
}
return DFS(0, 1);
}
}<file_sep>public class Solution {
public int LongestSubarray(int[] nums, int limit) {
var max = new LinkedList<int>();
var min = new LinkedList<int>();
var lo = 0;
var hi = 0;
var res = 1;
while(hi < nums.Length){
var v = nums[hi];
while(max.Any() && max.Last.Value < v)
max.RemoveLast();
max.AddLast(v);
while(min.Any() && min.Last.Value > v)
min.RemoveLast();
min.AddLast(v);
while(max.First.Value - min.First.Value > limit){
if(nums[lo] == max.First.Value)
max.RemoveFirst();
if(nums[lo] == min.First.Value)
min.RemoveFirst();
lo++;
}
res = Math.Max(res, hi-lo+1);
hi++;
}
return res;
}
}<file_sep>
/*
Let's say nums looks like this: [12, 13, 14, 15, 16, 17, 18, 19, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
Because it's not fully sorted, we can't do normal binary search. But here comes the trick:
If target is let's say 14, then we adjust nums to this, where "inf" means infinity:
[12, 13, 14, 15, 16, 17, 18, 19, inf, inf, inf, inf, inf, inf, inf, inf, inf, inf, inf, inf]
If target is let's say 7, then we adjust nums to this:
[-inf, -inf, -inf, -inf, -inf, -inf, -inf, -inf, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]
If nums[mid] and target are "on the same side" of nums[0], we just take nums[mid]. Otherwise we use -infinity or +infinity as needed.
*/
public class Solution {
public int Search(int[] nums, int target) {
var l = 0;
var h = nums.Length-1;
var sub = target < nums[0] ? int.MinValue : int.MaxValue;
while(l <= h){
var mid = l + (h-l)/2;
var midVal = nums[mid] < nums[0] == target < nums[0]
? nums[mid]
: sub;
if (midVal == target)
return mid;
if (target > midVal)
l = mid+1;
else
h = mid-1;
}
return -1;
}
}
public class Solution {
public int Search(int[] nums, int target) {
var l = 0;
var h = nums.Length-1;
while(l <= h){
var mid = l + (h-l)/2;
var midVal = nums[mid];
if (midVal == target)
return mid;
if (target > midVal)
{
if (midVal <= nums[h] && target > nums[h]){
h = mid-1;
} else {
l = mid+1;
}
}
if (target < midVal)
{
if (midVal >= nums[l] && target < nums[l]){
l = mid+1;
} else {
h = mid-1;
}
}
}
return -1;
}
}<file_sep>public class Solution {
public int RemoveStones(int[][] stones) {
var dsu = new DSU();
var colsOffcet = 10000;
foreach(var stone in stones){
dsu.Union(stone[0], stone[1] + colsOffcet);
}
var sets = new HashSet<int>();
foreach(var stone in stones){
sets.Add(dsu.Find(stone[0]).Val);
}
return stones.Length - sets.Count;
}
public class DSU{
private Dictionary<int, Node> map = new Dictionary<int, Node>();
public Node Create(int v){
if (!map.TryGetValue(v, out var node))
node = map[v] = new Node(v);
return node;
}
public void Union(int a, int b){
var parentA = Find(Create(a));
var parentB = Find(Create(b));
if (parentA != parentB)
parentB.Parent = parentA;
}
public Node Find(int v){
return Find(map[v]);
}
public Node Find(Node n){
return n.Parent == n ? n : Find(n.Parent);
}
}
public class Node{
public Node Parent;
public int Val;
public Node(int val){
Parent = this;
Val = val;
}
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public IList<TreeNode> FindDuplicateSubtrees(TreeNode root) {
var set = new Dictionary<string, bool>();
var res = new List<TreeNode>();
string Lookup(TreeNode node){
if(node == null)
return "#";
var id = node.val + "," + Lookup(node.left) + "," + Lookup(node.right);
if(set.TryGetValue(id, out var used)){
if(!used){
res.Add(node);
set[id] = true;
}
} else {
set[id] = false;
}
return id;
}
Lookup(root);
return res;
}
}<file_sep>public class Solution {
public int[] Intersect(int[] nums1, int[] nums2) {
if(nums1.Length > nums2.Length)
return Intersect(nums2, nums1);
Dictionary<int, int> toMap(int[] nums){
var map = new Dictionary<int, int>();
foreach(var n in nums){
if(map.ContainsKey(n))
map[n]++;
else
map[n] = 1;
}
return map;
}
var map1 = toMap(nums1);
var map2 = toMap(nums2);
var res = new List<int>();
foreach(var kv in map1){
if(map2.TryGetValue(kv.Key, out var count))
res.AddRange(Enumerable.Repeat(kv.Key, Math.Min(kv.Value, count)));
}
return res.ToArray();
}
}<file_sep>
public class Solution {
public Node Insert(Node head, int insertVal) {
if(head == null){
head = new Node(insertVal);
head.next = head;
return head;
}
var node = head;
var inserted = false;
var maxNode = head;
do{
var next = node.next;
if(insertVal >= node.val && insertVal < next.val){
node.next = new Node(insertVal, next);
inserted = true;
break;
}
if(node.val >= maxNode.val)
maxNode = node;
node = next;
}while(node != head);
if(!inserted){
maxNode.next = new Node(insertVal, maxNode.next);
}
return head;
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int x) { val = x; }
* }
*/
public class Solution {
public bool IsValidBST(TreeNode root) {
return EnsureInterval(root, null, null);
}
private bool EnsureInterval(TreeNode n, int? min, int? max)
{
if (n == null)
return true;
if ((min == null || n.val > min.Value) && (max == null || n.val < max.Value) )
return EnsureInterval(n.left, min, n.val) && EnsureInterval(n.right, n.val, max);
return false;
}
}<file_sep>public class Solution {
int ans = int.MaxValue;
public int TilingRectangle(int n, int m) {
dfs(0, 0, new bool[n,m], 0);
return ans;
}
// (r, c) is the starting point for selecting a square
// rect records the status of current rectangle
// cnt is the number of squares we have covered
private void dfs(int r, int c, bool[,] rect, int cnt) {
var n = rect.GetLength(0);
var m = rect.GetLength(1);
// optimization 1: The current cnt >= the current answer
if (cnt >= ans)
return;
// Successfully cover the whole rectangle
if (r == n) {
ans = cnt;
return;
}
// Successfully cover the area [0, ..., n][0, ..., c] => Move to next row
if (c == m) {
dfs(r+1, 0, rect, cnt);
return;
}
// If (r, c) is already covered => move to next point (r, c+1)
if (rect[r,c]) {
dfs(r, c+1, rect, cnt);
return;
}
// Try all the possible size of squares starting from (r, c)
for (int k = Math.Min(n-r, m-c); k >= 1 && isAvailable(rect, r, c, k); k--) {
cover(rect, r, c, k);
dfs(r, c+k, rect, cnt+1);
uncover(rect, r, c, k);
}
}
// Check if the area [r, ..., r+k][c, ..., c+k] is alreadc covered
private bool isAvailable(bool[,] rect, int r, int c, int k) {
for (int i = 0; i < k; i++){
for(int j = 0; j < k; j++){
if(rect[r+i,c+j])
return false;
}
}
return true;
}
// Cover the area [r, ..., r+k][c, ..., c+k] with a k * k square
private void cover(bool[,] rect, int r, int c, int k) {
for(int i = 0; i < k; i++){
for(int j = 0; j < k; j++){
rect[r+i,c+j] = true;
}
}
}
// Uncover the area [r, ..., r+k][c, ..., c+k]
private void uncover(bool[,] rect, int r, int c, int k) {
for(int i = 0; i < k; i++){
for(int j = 0; j < k; j++){
rect[r+i,c+j] = false;
}
}
}
}<file_sep>public class Solution {
public IList<int> FindClosestElements(int[] arr, int k, int x) {
var lo = 0;
var hi = arr.Length-k-1;
while(lo <= hi){
var mid = lo + (hi-lo)/2;
if(x - arr[mid] <= arr[mid + k] - x)
hi = mid - 1;
else
lo = mid + 1;
}
var res = new List<int>();
hi = lo+k;
while(lo < hi)
res.Add(arr[lo++]);
return res;
}
}
public class Solution {
public IList<int> FindClosestElements(int[] arr, int k, int x) {
var res = new List<int>();
if(k <= 0 || arr.Length == 0)
return res;
if(x <= arr[0]){
for(int i = 0; i < k; i++)
res.Add(arr[i]);
return res;
}
if(x >= arr[arr.Length-1]){
for(int i = arr.Length-k; i < arr.Length; i++)
res.Add(arr[i]);
return res;
}
var lo = 0;
var hi = arr.Length-1;
while(lo < hi){
var mid = lo + (hi-lo)/2;
if(arr[mid] == x){
hi = mid;
break;
}
if(arr[mid] > x)
hi = mid;
else
lo = mid + 1;
}
lo = hi - 1;
for(int i = 0; i < k; i++){
if(lo < 0){
hi++;
continue;
}
if(hi >= arr.Length){
lo--;
continue;
}
var a = arr[lo];
var b = arr[hi];
var d = Math.Abs(a - x) - Math.Abs(b - x);
if(d < 0 || d == 0 && a < b)
lo--;
else
hi++;
}
lo++;
hi--;
while(lo <= hi)
res.Add(arr[lo++]);
return res;
}
}<file_sep>public class Solution {
public IList<string> LetterCombinations(string digits) {
var arr = new []{
new []{'a','b','c'},
new []{'d','e','f'},
new []{'g','h','i'},
new []{'j','k','l'},
new []{'m','n','o'},
new []{'p','q','r','s'},
new []{'t','u','v'},
new []{'w','x','y','z'}
};
var res = new List<string>();
for(int i = digits.Length-1; i >= 0; i--){
var idx = digits[i] - '0' - 2;
if(res.Count == 0){
res = arr[idx].Select(c => c.ToString()).ToList();
continue;
}
var newRes = new List<string>();
foreach(var c in arr[idx]){
for(int j = 0; j < res.Count; j++)
newRes.Add(c + res[j]);
}
res = newRes;
}
return res;
}
}<file_sep>public class Solution {
public int MinDeletions(string s) {
var frq = new int[26];
foreach(var c in s)
frq[c-'a']++;
Array.Sort(frq);
var res = 0;
for(int i = frq.Length-2; i >= 0 && frq[i] > 0; i--){
var d = frq[i] - frq[i+1];
if(d >= 0){
d = frq[i] == d ? d : d+1;
frq[i] -= d;
res += d;
}
}
return res;
}
}<file_sep>public class Solution {
public string LongestCommonPrefix(string[] strs) {
var sb = new StringBuilder();
if(strs.Length == 0)
return "";
var pos = 0;
while(pos < strs[0].Length){
var c = strs[0][pos];
foreach(var str in strs){
if(pos >= str.Length || str[pos] != c)
return sb.ToString();
}
sb.Append(c);
pos++;
}
return sb.ToString();
}
}<file_sep>public class Solution {
public int CoinChange(int[] coins, int amount) {
return CoinChange(coins, amount, new int[amount]);
}
private int CoinChange(int[] coins, int amount, int[] memo){
if (amount == 0)
return 0;
if (memo[amount-1] != 0)
return memo[amount-1];
var min = int.MaxValue;
for(var i = 0; i < coins.Length; i++){
if(coins[i] > amount)
continue;
var res = CoinChange(coins, amount-coins[i], memo);
if(res >= 0 && res < min)
min = res;
}
min = min == int.MaxValue ? -1 : min+1;
memo[amount-1] = min;
return min;
}
}<file_sep>
public class Solution {
public ListNode ReverseKGroup(ListNode head, int k) {
if(k==1)
return head;
/*
1-2-3-4 2
2-1-4-3
*/
ListNode prevSubHead = null;
var subHead = head; // 1
while(subHead != null){ // t
var nextHead = subHead; // null
for(int i = 0; i < k; i++){ // 1
if(nextHead == null)
return head;
nextHead = nextHead.next;
}
// !!! list integrity
ListNode prev = nextHead; // null
var curr = subHead; // 3
while(curr != null && curr != nextHead){ // t
var t = curr.next; // null
curr.next = prev; // 2 - 1 - | 4 - 3 - null
prev = curr; // 3
curr = t; // 4
}
if(prevSubHead == null){
head = prev;
} else{
prevSubHead.next = prev;
}
prevSubHead = subHead;
subHead = nextHead; // 3
}
return head;
}
}<file_sep>public class Solution {
public int OrangesRotting(int[][] grid) {
var rows = grid.Length;
var cols = grid[0].Length;
var q = new Queue<(int, int)>();
var freshCount = 0;
for(int r = 0; r < rows; r++){
for(int c = 0; c < cols; c++){
if(grid[r][c] == 1)
freshCount++;
else if(grid[r][c] == 2)
q.Enqueue((r,c));
}
}
var dir = new []{(1,0), (0,1), (-1,0), (0,-1)};
var minutes = 0;
while(q.Any() && freshCount > 0){
var count = q.Count;
for(int i = 0; i < count; i++){
var (row, col) = q.Dequeue();
foreach(var (rd, cd) in dir){
var r = row+rd;
var c = col+cd;
if(r >= 0 && r < rows && c >= 0 && c < cols && grid[r][c] == 1){
q.Enqueue((r,c));
grid[r][c] = 2;
freshCount--;
}
}
}
minutes++;
}
return freshCount > 0 ? -1 : minutes;
}
}<file_sep>public class Solution {
public string StoneGameIII(int[] stoneValue) {
var n = stoneValue.Length;
var maxScore = new int[n];
maxScore[n-1] = stoneValue[n-1];
for(int i = n-2; i >= 0; i--)
maxScore[i] = maxScore[i+1] + stoneValue[i];
var memo = new int?[n];
int DFS(int pile){
if(pile >= n)
return 0;
if(memo[pile].HasValue)
return memo[pile].Value;
var min = int.MaxValue;
for(var i=1; i <= 3; i++){
min = Math.Min(min, DFS(pile+i));
}
memo[pile] = maxScore[pile] - min;
return memo[pile].Value;
}
var score = DFS(0)*2;
return score == maxScore[0]
? "Tie"
: score > maxScore[0]
? "Alice"
: "Bob";
}
}<file_sep>public class Solution {
public string MostCommonWord(string paragraph, string[] banned) {
var maxCount = 0;
string res = null;
var frq = new Dictionary<string, int>();
var bannedSet = new HashSet<string>(banned);
foreach(var w in paragraph.Split(" !?',;.".ToCharArray())){
if(string.IsNullOrEmpty(w))
continue;
var word = w.ToLower();
if(frq.ContainsKey(word))
frq[word]++;
else
frq[word] = 1;
if(!banned.Contains(word) && frq[word] > maxCount){
maxCount = frq[word];
res = word;
}
}
return res;
}
}<file_sep>/*
// Definition for a Node.
public class Node {
public int val;
public Node next;
public Node random;
public Node(int _val) {
val = _val;
next = null;
random = null;
}
}
*/
public class Solution {
public Node CopyRandomList(Node head) {
var map = new Dictionary<Node, Node>();
var curr = head;
Node prev = null;
while(curr != null){
var newNode = new Node(curr.val);
if(prev != null)
prev.next = newNode;
map[curr] = newNode;
curr = curr.next;
prev = newNode;
}
curr = head;
while(curr != null){
if(curr.random != null){
map[curr].random = map[curr.random];
}
curr = curr.next;
}
return head != null ? map[head] : null;
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public IList<string> BinaryTreePaths(TreeNode root) {
var res = new List<string>();
if(root == null)
return res;
var q = new Queue<(TreeNode, string)>();
q.Enqueue((root, ""));
while(q.Any()){
var (node, path) = q.Dequeue();
if(path != "")
path += "->";
path += node.val;
if(node.left == null && node.right == null)
res.Add(path);
else {
if(node.left != null)
q.Enqueue((node.left, path));
if(node.right != null)
q.Enqueue((node.right, path));
}
}
return res;
}
}<file_sep>public class Solution {
/*
ab
adc
*/
public string AlienOrder(string[] words) {
var g = new Dictionary<char, HashSet<char>>(); // c[d] d[f]
var indegree = new Dictionary<char, int>(); // c0 d1 f1
foreach(var word in words)
foreach(var c in word)
indegree[c] = 0;
for(int i = 1; i < words.Length; i++){ // f
var w1 = words[i-1]; // abd
var w2 = words[i]; // abf
var pos = 0; // 2
while(pos < w1.Length && pos < w2.Length){
var a = w1[pos]; // d
var b = w2[pos]; // f
if(a != b){ // t
if(!g.ContainsKey(a) || !g[a].Contains(b)){
if(!g.ContainsKey(a))
g[a] = new HashSet<char>();
g[a].Add(b);
indegree[b]++;
}
break;
}
pos++;
}
// w1 should not be longer!
if(pos == w2.Length && w2.Length != w1.Length) // adc ab
return "";
}
var q = new Queue<char>(); // d
foreach(var kv in indegree){
if(kv.Value == 0)
q.Enqueue(kv.Key);
}
var sb = new StringBuilder(); // cd
// c[d] d[f]
// c0 d0 f0
while(q.Any()){
var c = q.Dequeue();
sb.Append(c);
if(!g.ContainsKey(c))
continue;
foreach(var nei in g[c]){
indegree[nei]--;
if(indegree[nei] == 0)
q.Enqueue(nei);
}
}
Console.WriteLine(sb.ToString());
return sb.Length == indegree.Count ? sb.ToString() : "";
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int x) { val = x; }
* }
*/
public class Codec {
// Encodes a tree to a single string.
public string serialize(TreeNode root) {
var sb = new StringBuilder();
serialize(root, sb);
return sb.ToString();
}
public void serialize(TreeNode node, StringBuilder sb) {
if(node == null)
return;
sb.Append(node.val).Append(' ');
serialize(node.left, sb);
serialize(node.right, sb);
}
// Decodes your encoded data to tree.
public TreeNode deserialize(string data) {
var q = new Queueu<int>();
foreach(var str in data.Split(' '))
q.Enqueue(int.Parse(str));
return deserialize(q, int.MinValue, int.MaxValue);
}
public TreeNode deserialize(Queue<int> q, int min, int max) {
if(!q.Any())
return null;
var n = q.Peek();
if(n < min && n > max)
return null;
q.Dequeue();
var node = new TreeNode(n);
node.left = deserialize(q, min, n);
node.right = deserialize(q, n, max);
return node;
}
}
// Your Codec object will be instantiated and called as such:
// Codec ser = new Codec();
// Codec deser = new Codec();
// String tree = ser.serialize(root);
// TreeNode ans = deser.deserialize(tree);
// return ans;<file_sep>public class Solution {
public double MyPow(double x, int n) {
var N = (long)n;
if(N < 0){
N *= -1;
x = 1/x;
}
double fastPow(double x, long n){
if(n == 0)
return 1;
var res = fastPow(x, n/2);
res *= res;
if(n % 2 == 1)
res *= x;
return res;
}
return fastPow(x, N);
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public int KthSmallest(TreeNode root, int k) {
var st = new Stack<TreeNode>();
void moveLeft(TreeNode node){
while(node != null){
st.Push(node);
node = node.left;
}
}
moveLeft(root);
while(st.Any()){
var node = st.Pop();
if(--k == 0)
return node.val;
if(node.right != null){
moveLeft(node.right);
}
}
return -1;
}
}<file_sep>public class Solution {
public bool CanFinish(int n, int[][] prerequisites) {
var inDegree = new int[n];
var g = new Dictionary<int, HashSet<int>>();
foreach(var edge in prerequisites){
var next = edge[0];
var prev = edge[1];
inDegree[next]++;
if(!g.ContainsKey(prev))
g[prev] = new HashSet<int>();
g[prev].Add(next);
}
var q = new Queue<int>();
for(int i = 0; i < n; i++){
if(inDegree[i] == 0)
q.Enqueue(i);
}
while(q.Any()){
var course = q.Dequeue();
if(g.ContainsKey(course)){
foreach(var next in g[course]){
inDegree[next]--;
if(inDegree[next] == 0)
q.Enqueue(next);
}
}
}
return !inDegree.Any(course => course > 0);
}
}<file_sep>public class Solution {
public string FindReplaceString(string S, int[] indexes, string[] sources, string[] targets) {
if(S == "")
return S;
var replacements = new int[S.Length];
for(int i = 0; i < indexes.Length; i++){
var pos = indexes[i];
var source = sources[i];
var k = 0;
while(k < source.Length && S[pos+k] == source[k])
k++;
if(k == source.Length){
replacements[pos] = i+1;
for(var j = 1; j < source.Length; j++)
replacements[pos+j] = -1;
}
}
var sb = new StringBuilder();
var ptr = 0;
for(var i = 0; i < replacements.Length; i++){
var idx = replacements[i];
if(idx != -1){
if (idx > 0){
foreach(var c in targets[idx-1])
sb.Append(c);
} else {
sb.Append(S[ptr]);
}
}
ptr++;
}
return sb.ToString();
}
}<file_sep>// 438, 3
public class Solution {
public string MinWindow(string s, string p) {
var res = "";
if(s.Length == 0 || p.Length > s.Length)
return res;
var frq = new Dictionary<char, int>();
foreach(var c in p)
frq[c] = frq.ContainsKey(c) ? frq[c] + 1 : 1;
var count = p.Length;
var lo = 0;
var hi = 0;
while(hi < s.Length){
var c = s[hi++];
if(frq.ContainsKey(c)){
frq[c]--;
if(frq[c] >= 0)
count--;
}
while(count == 0 && lo < hi){
if(res == "" || hi - lo < res.Length)
res = s.Substring(lo, hi-lo);
c = s[lo++];
if(frq.ContainsKey(c)){
frq[c]++;
if(frq[c] > 0)
count++;
}
}
}
return res;
}
}<file_sep>public class FileSystem {
private Node fs;
public FileSystem() {
fs = new Node{Name = "/"};
}
public IList<string> Ls(string path) {
var dir = Navigate(path);
var ls = new List<string>();
if(dir.IsFile){
ls.Add(dir.Name);
return ls;
}
if(dir.Children == null)
return ls;
foreach(var kv in dir.Children)
ls.Add(kv.Key);
return ls;
}
public void Mkdir(string path) {
Navigate(path);
}
public void AddContentToFile(string filePath, string content) {
var f = Navigate(filePath);
f.IsFile = true;
f.Content = f.Content == null ? content : f.Content + content;
}
public string ReadContentFromFile(string filePath) {
var f = Navigate(filePath);
return f.Content;
}
private Node Navigate(string path){
var cur = fs;
foreach(var name in path.Split('/'))
if(!string.IsNullOrEmpty(name))
cur = cur.GetAddChild(name);
return cur;
}
private class Node{
public string Name;
public SortedDictionary<string, Node> Children;
public string Content;
public bool IsFile;
public Node GetAddChild(string name){
if(Children == null)
Children = new SortedDictionary<string, Node>();
if(!Children.ContainsKey(name))
Children[name] = new Node{Name = name};
return Children[name];
}
}
}
<file_sep>public class Solution {
public string Encode(string s) {
return Encode(s, new Dictionary<string, string>());
}
public string Encode(string s, Dictionary<string, string> memo){
var n = s.Length;
if(n <= 4)
return s;
if(memo.ContainsKey(s))
return memo[s];
string res = null;
for(var i=1; i <= n/2; i++){
var sub = s.Substring(0, i);
var repeatCount = CountRepetitions(s, sub);
for(var j = 1; j <= repeatCount; j++){
var head = j == 1 ? sub : $"{j}[{Encode(sub, memo)}]";
if(res != null && head.Length >= res.Length)
break;
var tail = Encode(s.Substring(i*j), memo);
if(res == null || head.Length+tail.Length < res.Length)
res = head+tail;
}
}
memo[s] = res;
return res;
}
private int CountRepetitions(string s, string sub){
var res = 1;
var n = sub.Length;
for(var i = n; i <= s.Length-n; i += n){
var j = 0;
while(j<n && s[i+j] == s[j])
j++;
if(j != n)
break;
res++;
}
return res;
}
}<file_sep>public class Solution {
public IList<int> MostSimilar(int n, int[][] roads, string[] names, string[] targetPath) {
var g = new List<int>[n];
var len = targetPath.Length;
foreach(var r in roads){
var a = r[0];
var b = r[1];
if(g[a] == null)
g[a] = new List<int>();
if(g[b] == null)
g[b] = new List<int>();
g[a].Add(b);
g[b].Add(a);
}
var end = targetPath[len-1];
var dp = new int[len, n][];
for(int j = 0; j < n; j++){
dp[len-1,j] = new []{ names[j]==end ? 0 : 1, -1}; // cost;next city
}
for(var i = len-1; i > 0; i--){
var currMin = int.MaxValue;
for(var j = 0; j < n; j++){
var city = dp[i,j];
if(city == null || city[0]-i >= currMin)
continue;
foreach(var nei in g[j]){
var cost = city[0] + (names[nei] == targetPath[i-1] ? 0 : 1);
if(dp[i-1, nei] == null || cost < dp[i-1, nei][0]){
dp[i-1, nei] = new []{cost, j};
currMin = Math.Min(currMin,cost);
}
}
}
}
var min = int.MaxValue;
var res = new int[len];
for(int j = 0; j < n; j++){
var cur = dp[0,j];
if(cur != null && cur[0] < min){
min = cur[0];
res[0] = j;
}
}
for(int i = 0; i < len-1; i++){
res[i+1] = dp[i,res[i]][1];
}
return res;
}
}<file_sep>public class Solution {
public string[] ReorderLogFiles(string[] logs) {
return logs
.Select(l => new Record(l))
.OrderBy(r => r)
.Select(r => r.Str)
.ToArray();
}
class Record : IComparable<Record>{
private bool isDigit;
private string[] split;
public string Str;
public Record(string str){
Str = str;
split = str.Split(' ', 2);
isDigit = split[1][0] <= '9';
}
public int CompareTo(Record other){
if(isDigit)
return other.isDigit ? 0 : 1;
if(other.isDigit)
return -1;
var res = split[1].CompareTo(other.split[1]);
return res == 0
? split[0].CompareTo(other.split[0])
: res;
}
}
}<file_sep>public class Solution {
public int LongestLine(int[][] M) {
var rows = M.Length;
if(rows == 0)
return 0;
var cols = M[0].Length;
var max = 0;
var dp = new int[cols, 3];
for(var r = 0; r < rows; r++){
var row = 0;
var prevDiag = 0;
for(var c = 0; c < cols; c++){
var col = 0;
var diag = 0;
var adiag = 0;
if(M[r][c] == 1){
row++;
col = dp[c,0] + 1;
diag = c != 0 ? prevDiag + 1 : 1;
adiag = c < cols-1 ? dp[c+1,2] + 1 : 1;
max = Math.Max(max , Math.Max(row, Math.Max(col, Math.Max(diag, adiag))));
} else {
row = 0;
}
dp[c, 0] = col;
// !!! prevent override
prevDiag = dp[c, 1];
dp[c, 1] = diag;
dp[c, 2] = adiag;
}
}
return max;
}
}<file_sep>public class Solution {
public IList<string> FindAllConcatenatedWordsInADict(string[] words) {
var res = new List<string>();
if(words.Length < 2)
return res;
var sorted = words.Where(w => w != null && w.Length > 0).OrderBy(w => w.Length).ToArray();
var minLen = sorted[0].Length;
var wordsSet = new HashSet<string>();
var maxLen = 0;
foreach(var word in sorted){
//if(word.Length >= minLen*2 && WordBreak(word, wordsSet, minLen, maxLen))
if(word.Length >= minLen*2 && WordBreak(word, wordsSet))
res.Add(word);
wordsSet.Add(word);
maxLen = Math.Max(maxLen, word.Length);
}
return res;
}
public bool WordBreak(string s, HashSet<string> set) {
var n = s.Length;
var memo = new bool[n+1];
memo[0] = true;
for(int i = 1; i<= n; i++){
for(int j = i-1; j >=0; j--){
if(memo[j] && set.Contains(s.Substring(j, i-j))){
memo[i] = true;
break;
}
}
}
return memo[n];
}
public bool WordBreak(string s, HashSet<string> words, int minLen, int maxLen) {
var memo = new List<bool?>[s.Length];
bool DFS(int idx){
if(memo[idx] != null)
return memo[idx];
var res = false;
var sb = new StringBuilder();
for(int i = idx; i < s.Length && i-idx+1 <= maxLen; i++){
sb.Append(s[i]);
if(i-idx+1 < minLen)
continue;
var word = sb.ToString();
if(words.Contains(word) && (i == s.Length-1 || DFS(i+1))){
res = true;
break;
}
}
memo[idx] = res;
return res;
}
return DFS(0);
}
}<file_sep>public class Solution {
public int PeakIndexInMountainArray(int[] arr) {
int lo = 0, hi = arr.Length - 1;
while (lo < hi) {
int mi = lo + (hi - lo) / 2;
if (arr[mi] < arr[mi + 1])
lo = mi + 1;
else
hi = mi;
}
return hi;
}
}<file_sep>public class Solution {
/*
a
ac
aa
aaa
aaaaaa
aaabaaa
aaabaaa
aaaaaaa
*/
public bool IsOneEditDistance(string s, string t) {
var lenS = s.Length; // 3
var lenT = t.Length; // 2
if(lenS != lenT && Math.Abs(lenS - lenT) != 1)
return false;
var edited = false;
var idxS = 0; // 1
var idxT = 0; // 1
while(idxS < lenS && idxT < lenT){ // t
if(s[idxS] == t[idxT]){ // t
idxS++;
idxT++;
} else {
if(edited) // f
return false;
edited = true;
if(lenS <= lenT) // f
idxT++;
if(lenT <= lenS) // t
idxS++;
}
}
return edited && idxS == lenS && idxT == lenT || !edited && Math.Abs(lenS - lenT) == 1;
}
}<file_sep>public class Solution {
public IList<string> RemoveInvalidParentheses(string s) {
var res = new List<string>();
dfs(s, res, false, 0, 0);
return res;
}
public static void dfs(String s, List<String> res, bool isReversed, int last_i, int last_j) {
var check = isReversed ? new char[]{')', '('} : new char[]{'(', ')'};
var count = 0;
var i = last_i;
for (; i < s.Length && count>= 0; i++) {
if (s[i] == check[0]) count++;
if (s[i] == check[1]) count--;
}
if (count >= 0) {
// no extra ')' is detected. We now have to detect extra '(' by reversing the string.
var reversed = new String(s.Reverse().ToArray());
if (!isReversed)
dfs(reversed, res, true, 0, 0);
else
res.Add(reversed);
}
else { // extra ')' is detected and we have to do something
i -= 1; // 'i-1' is the index of abnormal ')' which makes count<0
for (int j = last_j; j <= i; j++) {
if (s[j] == check[1] && (j == last_j || s[j-1] != check[1])) {
dfs(s.Remove(j,1), res, isReversed, i, j);
}
}
}
}
}<file_sep>// quick select
public class Solution {
public int[][] KClosest(int[][] points, int k) {
var n = points.Length;
if(n == k)
return points;
var res = new int[k][];
int distance(int[] p) => p[0]*p[0] + p[1]*p[1];
void swap(int a, int b){
if(a == b) return;
var t = points[a];
points[a] = points[b];
points[b] = t;
}
int partition(int lo, int hi){
var pivot = distance(points[hi]);
for(int i = lo; i < hi; i++){
if(distance(points[i]) < pivot)
swap(lo++, i);
}
swap(lo, hi);
return lo;
}
var lo = 0;
var hi = n-1;
var mid = -1;
while(lo < hi && mid != k){
mid = partition(lo, hi);
if(mid > k)
hi = mid-1;
else
lo = mid+1;
}
Array.Copy(points, res, k);
return res;
}
}<file_sep>/*
// Definition for a Node.
public class Node {
public int val;
public Node left;
public Node right;
public Node next;
public Node() {}
public Node(int _val) {
val = _val;
}
public Node(int _val, Node _left, Node _right, Node _next) {
val = _val;
left = _left;
right = _right;
next = _next;
}
}
*/
public class Solution {
public Node Connect(Node root) {
if(root == null)
return null;
var left = root;
while(left != null){
var parent = left;
left = parent.left;
while(parent != null){
parent.left.next = parent.right;
if(parent.next != null){
parent.right.next = parent.next.left;
parent = parent.next;
}
}
return root;
}
}<file_sep>public class Solution {
public string NextClosestTime(string time) {
var nums = new int[]{
time[0] - '0',
time[1] - '0',
time[3] - '0',
time[4] - '0'
};
var tmins = (nums[0]*10+nums[1])*60+nums[2]*10+nums[3];
var res = new int[4];
var diff = int.MaxValue;
foreach(var n1 in nums.Where(n => n < 3))
foreach(var n2 in nums.Where(n => n1*10+n < 24))
foreach(var n3 in nums.Where(n => n < 6))
foreach(var n4 in nums.Where(n => n3*10+n < 60)){
if (n1*10+n2 < 24 && n3*10+n4 < 60){
var mins = (n1*10+n2)*60+n3*10+n4;
if(mins <= tmins)
mins = mins + 60 * 24;
if(mins - tmins < diff){
res[0]=n1;
res[1]=n2;
res[2]=n3;
res[3]=n4;
diff = mins - tmins;
}
}
}
return $"{res[0]}{res[1]}:{res[2]}{res[3]}";
}
static IEnumerable<ValueTuple<int,int,int,int>> DoPermute(int[] nums, int start, int end)
{
if (start == end)
{
yield return (nums[0], nums[1], nums[2], nums[3]);
}
else
{
for (var i = start; i <= end; i++)
{
Swap(ref nums[start], ref nums[i]);
foreach (var r in DoPermute(nums, start + 1, end))
yield return r;
Swap(ref nums[start], ref nums[i]);
}
}
}
static void Swap(ref int a, ref int b)
{
var temp = a;
a = b;
b = temp;
}
}<file_sep>public class Solution {
public int[] AssignBikes(int[][] workers, int[][] bikes) {
var lenW = workers.Length;
var lenB = bikes.Length;
var res = new int[lenW];
var buckets = new List<ValueTuple<int, int>>[2000];
for(var w = 0; w < lenW; w++){
for(var b = 0; b < lenB; b++){
var dist = Math.Abs(bikes[b][0] - workers[w][0]) + Math.Abs(bikes[b][1] - workers[w][1]);
if (buckets[dist] == null){
buckets[dist] = new List<ValueTuple<int,int>>{(w, b)};
} else {
buckets[dist].Add((w,b));
}
}
}
var left = lenW;
for(int i = 0; i < buckets.Length && left > 0; i++){
var list = buckets[i];
if(list == null)
continue;
foreach((var w, var b) in list){
if(workers[w] != null && bikes[b] != null){
res[w] = b;
workers[w] = null;
bikes[b] = null;
left--;
}
}
}
return res;
}
}<file_sep>public class Solution {
public IList<string> WordBreak(string s, IList<string> wordDict) {
var words = new HashSet<string>();
var maxLen = 0;
var minLen = int.MaxValue;
var memo = new List<string>[s.Length];
foreach(var w in wordDict){
maxLen = Math.Max(maxLen, w.Length);
minLen = Math.Min(minLen, w.Length);
words.Add(w);
}
List<string> DFS(int idx){
if(memo[idx] != null)
return memo[idx];
var res = new List<string>();
var sb = new StringBuilder();
for(int i = idx; i < s.Length && i-idx+1 <= maxLen; i++){
sb.Append(s[i]);
if(i-idx+1 < minLen)
continue;
var word = sb.ToString();
if(words.Contains(word)){
if(i == s.Length-1){
res.Add(word);
} else {
foreach(var str in DFS(i+1))
res.Add(word + " " + str);
}
}
}
memo[idx] = res;
return res;
}
return DFS(0);
}
}<file_sep>public class Solution {
public int NumMatchingSubseq(string S, string[] words) {
var res = 0;
var n = words.Length;
var buckets = new List<Wrapper>[26];
foreach(var word in words){
var w = new Wrapper(word);
var idx = w.Head - 'a';
if(buckets[idx] == null)
buckets[idx] = new List<Wrapper>(5000);
buckets[idx].Add(w);
}
for(int i = 0; i < S.Length; i++){
var idx = S[i]-'a';
var bucket = buckets[idx];
if(bucket == null || !bucket.Any())
continue;
var newBucket = new List<Wrapper>();
foreach(var w in bucket){
w.ptr += 1;
if(w.Head == -1){
res++;
continue;
}
if(w.Head == S[i])
newBucket.Add(w);
else{
if(buckets[w.Head - 'a'] == null)
buckets[w.Head - 'a'] = new List<Wrapper>(5000);
buckets[w.Head - 'a'].Add(w);
}
}
buckets[idx] = newBucket;
}
return res;
}
class Wrapper{
string s;
public int ptr;
public Wrapper(string s){
this.s = s;
}
public int Head => ptr < s.Length ? s[ptr] : -1;
}
}<file_sep>public class Solution {
public bool IsPossibleDivide(int[] hand, int W) {
var n = hand.Length;
if(n % W != 0)
return false;
var heap = new SortedDictionary<int, int>();
for(int i = 0; i < n; i++){
if(heap.ContainsKey(hand[i]))
heap[hand[i]] += 1;
else
heap[hand[i]] = 1;
}
for(int i = 0; i < n / W; i++){
var min = heap.First().Key;
for(int j = 0; j < W; j++){
if(!heap.ContainsKey(min+j))
return false;
if(heap[min+j] > 1)
heap[min+j] -= 1;
else
heap.Remove(min+j);
}
}
return true;
}
}<file_sep>public class Solution {
public int MaxLength(IList<string> arr) {
var n = arr.Count;
var dp = new List<(int, int)>();
dp.Add((0,0));
var max = 0;
for(int i = 0; i < n; i++){
var str = arr[i];
var mask = 0;
foreach(var c in str){
var b = 1 << (c-'a');
if((b | mask) != (b ^ mask)){
mask = -1;
break;
}
mask |= b;
}
if(mask == -1)
continue;
for(int j = dp.Count-1; j >= 0; j--){
var (m,l) = dp[j];
if((m & mask) == 0){
var len = str.Length + l;
dp.Add((m | mask, len));
max = Math.Max(max, len);
}
}
}
return max;
}
}<file_sep>public class Solution {
public int SumSubarrayMins(int[] A) {
int MOD = 1000000007;
var res = 0;
var mins = new Stack<ValueTuple<int, int>>();
var sum = 0;
for(int i=0; i < A.Length; i++){
var v = A[i];
var count = 1;
while(mins.Count > 0)
{
(var vPrev, var countPrev) = mins.Peek();
if(vPrev<v)
break;
count += countPrev;
mins.Pop();
sum -= (vPrev*countPrev);
}
mins.Push((v, count));
sum += v*count;
res += sum;
res %= MOD;
}
return res;
}
}<file_sep>public class Solution {
public int[] PrisonAfterNDays(int[] cells, int N) {
int calcState(int[] a){
var b = 0;
for(int i = 0; i < a.Length; i++){
b <<= 1;
b |= a[i];
}
return b;
}
var visited = new Dictionary<int, int>();
visited[calcState(cells)] = 0;
var daysCount = 1;
while(N > 0){
var curCells = new int[cells.Length];
for(int i = 1; i < cells.Length-1; i++)
curCells[i] = cells[i-1] != cells[i+1] ? 0 : 1;
if(visited != null){
var curState = calcState(curCells);
if(visited.ContainsKey(curState)){
var cycleLength = daysCount-visited[curState];
N %= cycleLength; // magic
visited = null;
} else {
visited[curState] = daysCount++;
}
}
if(N > 0)
cells = curCells;
N--;
}
return cells;
}
}<file_sep>public class Solution {
public double[] CalcEquation(IList<IList<string>> equations, double[] values, IList<IList<string>> queries) {
var graph = new Dictionary<string, Dictionary<string, double>>();
for (var i = 0; i < equations.Count; i++){
var dividend = equations[i][0];
var divisor = equations[i][1];
var quotent = values[i];
if (!graph.TryGetValue(dividend, out var d1)){
d1 = new Dictionary<string, double>();
graph.Add(dividend, d1);
}
if (!graph.TryGetValue(divisor, out var d2)){
d2 = new Dictionary<string, double>();
graph.Add(divisor, d2);
}
d1.Add(divisor, quotent);
d2.Add(dividend, 1/quotent);
}
var res = new double[queries.Count];
for(var i = 0; i < queries.Count; i++){
var start = queries[i][0];
var end = queries[i][1];
if (!graph.ContainsKey(start) || !graph.ContainsKey(end)){
res[i] = -1;
continue;
}
if(start == end){
res[i] = 1;
continue;
}
res[i] = -1;
var visited = new HashSet<string>();
res[i] = DFS(graph, start, end, visited, 1);
/* DFS
var q = new Queue<ValueTuple<string, double>>();
q.Enqueue((start, 1.0));
while(q.Count > 0){
(string node, double sum) = q.Dequeue();
visited.Add(node);
var nodes = graph[node];
if (nodes.TryGetValue(end, out var val)){
res[i] = sum*val;
break;
}
foreach(var kv in nodes){
if(visited.Contains(kv.Key))
continue;
q.Enqueue((kv.Key, sum*kv.Value));
}
}
*/
}
return res;
}
private double DFS(Dictionary<string, Dictionary<string, double>> graph, string curr, string target, HashSet<string> visited, double sum){
var nodes = graph[curr];
if (nodes.TryGetValue(target, out var val)){
return sum * val;
}
visited.Add(curr);
var ret = -1.0;
foreach(var kv in nodes.Where(n => !visited.Contains(n.Key))){
ret = DFS(graph, kv.Key, target, visited, sum*kv.Value);
if(ret > -1)
break;
}
//visited.Remove(curr);
return ret;
}
}<file_sep>public class Solution {
/*
2
aabaccc
*/
public int LengthOfLongestSubstringKDistinct(string s, int k) {
if(k==0)
return 0;
int[] frq = new int[256];
int count = 0;
var lo = 0;
var res = 0;
for (int hi = 0; hi < s.Length; hi++) {
if (frq[s[hi]]++ == 0)
count++;
if (count > k) {
while (--frq[s[lo++]] > 0);
count--;
}
res = Math.Max(res, hi - lo + 1);
}
return res;
}
}<file_sep>public class MedianFinder {
SortedDictionary<int, int> lo;
SortedDictionary<int, int> hi;
int countLo;
int countHi;
public MedianFinder() {
lo = new SortedDictionary<int, int>();
hi = new SortedDictionary<int, int>(new DescComparer());
}
public void AddNum(int num) {
Add(lo, num, ref countLo);
var top = RemoveTop(lo, ref countLo);
Add(hi, top, ref countHi);
while(countHi > countLo){
top = RemoveTop(hi, ref countHi);
Add(lo, top, ref countLo);
}
}
public double FindMedian() {
var isOdd = (countLo+countHi)%2 == 1;
return isOdd
? lo.First().Key
: ((double)lo.First().Key + hi.First().Key) / 2;
}
private void Add(SortedDictionary<int, int> d, int num, ref int count){
if(d.ContainsKey(num))
d[num] += 1;
else
d[num] = 1;
count++;
}
private int RemoveTop(SortedDictionary<int, int> d, ref int count){
var top = d.First();
if(top.Value == 1)
d.Remove(top.Key);
else
d[top.Key] -= 1;
count--;
return top.Key;
}
class DescComparer : IComparer<int>{
public int Compare(int a, int b) {
return b-a;
}
}
}<file_sep>public class Solution {
public bool CheckIfExist(int[] arr) {
var set = new HashSet<int>();
foreach(var n in arr){
if(set.Contains(n*2) || (n%2 == 0 && set.Contains(n/2)))
return true;
set.Add(n);
}
return false;
}
}<file_sep>public class Solution {
public int MinKBitFlips(int[] A, int K) {
var q = new Queue<int>();
var n = A.Length;
var flips = 0;
for(int i = 0; i < n; i++){
if(A[i] == 0){
if(q.Count%2 == 0){
q.Enqueue(i+K-1);
flips++;
}
} else {
if(q.Any() && q.Count%2 == 1){
q.Enqueue(i+K-1);
flips++;
}
}
if(q.Any() && q.Peek() <= i)
q.Dequeue();
}
return q.Any() ? -1: flips;
}
}<file_sep>public class Solution {
public int Divide(int dividend, int divisor) {
if(dividend == int.MinValue && divisor == -1)
return int.MaxValue;
uint a = dividend >= 0 ? (uint)dividend : (uint)(-(int)(dividend+1))+1;
uint b = divisor >= 0 ? (uint)divisor : (uint)(-(int)(divisor+1))+1;
var res = 0;
for(int exp = 31; exp >= 0 && a >= b; exp--){
if(a >> exp >= b){
a -= b << exp;
res += 1 << exp;
}
}
return dividend > 0 == divisor > 0 ? res : -res;
}
}<file_sep> public class Solution
{
public bool BackspaceCompare(string S, string T)
{
var sCurrent = S.Length - 1;
var tCurrent = T.Length - 1;
while (sCurrent >= 0 && tCurrent >= 0)
{
PeekNextIndex(S, ref sCurrent);
PeekNextIndex(T, ref tCurrent);
if (sCurrent < 0 || tCurrent < 0)
return sCurrent == tCurrent;
if (S[sCurrent] != T[tCurrent])
return false;
sCurrent--;
tCurrent--;
}
PeekNextIndex(S, ref sCurrent);
PeekNextIndex(T, ref tCurrent);
return sCurrent == tCurrent;
}
private static void PeekNextIndex(string str, ref int current)
{
var skip = 0;
while (current >= 0)
{
if (str[current] == '#')
{
skip++;
}
else
{
if (skip > 0)
skip--;
else
return;
}
current--;
}
}
}<file_sep>/**
* // This is BinaryMatrix's API interface.
* // You should not implement it, or speculate about its implementation
* class BinaryMatrix {
* public int Get(int row, int col) {}
* public IList<int> Dimensions() {}
* }
*/
class Solution {
public int LeftMostColumnWithOne(BinaryMatrix binaryMatrix) {
var mat = binaryMatrix;
var rows = mat.Dimensions()[0];
var cols = mat.Dimensions()[1];
var row = 0;
var col = cols-1;
while(row < rows){
while(col >= 0 && mat.Get(row, col) == 1){
if (col-- == 0)
break;
}
row++;
}
return col == cols-1 ? -1 : col+1;
}
}<file_sep>public class Solution {
public int Reverse(int x) {
long result = 0;
var sign = x < 0 ? -1 : 1;
x *= sign;
while(x > 0){
result *= 10;
result += x % 10;
x /= 10;
if (result > int.MaxValue)
return 0;
}
return (int)result*sign;
}
}<file_sep>public class Solution {
public IList<IList<int>> CriticalConnections(int n, IList<IList<int>> connections) {
var g = new IList<IList<int>>[n];
foreach(var conn in connections){
if(g[conn[0]] == null)
g[conn[0]] = new List<IList<int>>();
if(g[conn[1]] == null)
g[conn[1]] = new List<IList<int>>();
g[conn[0]].Add(conn);
g[conn[1]].Add(conn);
}
var strongConnections = new HashSet<IList<int>>();
var dfsRanks = new int[n];
// to eliminate 1-1 = 0 on parent check
DFS(g, strongConnections, dfsRanks, 2, 0);
var res = new List<IList<int>>();
foreach(var conn in connections)
if(!strongConnections.Contains(conn))
res.Add(conn);
return res;
}
int DFS(IList<IList<int>>[] g, HashSet<IList<int>> conns, int[] ranks, int rank, int nodeIdx){
if(ranks[nodeIdx] > 0)
return ranks[nodeIdx];
ranks[nodeIdx] = rank;
var minDfsRank = rank;
foreach(var nei in g[nodeIdx]){
var neiIdx = nei[0] == nodeIdx ? nei[1] : nei[0];
// parent
if(ranks[neiIdx] == rank - 1)
continue;
var dfsRank = DFS(g, conns, ranks, rank+1, neiIdx);
if(dfsRank <= rank)
conns.Add(nei);
minDfsRank = Math.Min(minDfsRank, dfsRank);
}
return minDfsRank;
}
}<file_sep>public class Solution {
public int MinDifficulty(int[] jobDifficulty, int d) {
return jobDifficulty.Length >= d
? DFS(jobDifficulty, 0, d, new int?[jobDifficulty.Length, d])
: -1;
}
public int DFS(int[] jd, int idx, int remaining, int?[,] memo){
if(memo[idx, remaining-1].HasValue)
return memo[idx, remaining].Value;
var max = int.MinValue;
var res = int.MaxValue;
for(int i = idx; i < jd.Length - remaining + 1; i++){
max = Math.Max(max, jd[i]);
res = remaining == 1 ? max : Math.Min(res, max + DFS(jd, i+1, remaining-1, memo));
}
memo[idx, remaining-1] = res;
return res;
}
}<file_sep>public class Solution {
public int RangeBitwiseAnd(int m, int n) {
if (m==0)
return 0;
var shift = 0;
while (m != n){
m >>= 1;
n >>= 1;
shift++;
}
return m << shift;
}
}<file_sep>public class Solution {
public IList<IList<int>> Combine(int n, int k) {
var res = new List<IList<int>>();
Combine(1, 0, n, k, res, new int[k]);
return res;
}
public void Combine(int cur, int pos, int n, int k, IList<IList<int>> res, int[] comb) {
if(pos == k){
var arr = new int[k];
Array.Copy(comb, arr, k);
res.Add(arr);
return;
}
for(int i = cur; i <= n; i++){
comb[pos] = i;
Combine(i+1, pos+1, n, k, res, comb);
}
}
}<file_sep>public class Logger {
private const int n = 10;
private ValueTuple<int, HashSet<string>>[] hist = new ValueTuple<int, HashSet<string>>[n];
/** Initialize your data structure here. */
public Logger() {
for(int i = 0; i < n; i++)
hist[i] = (i, new HashSet<string>());
}
/** Returns true if the message should be printed in the given timestamp, otherwise returns false.
If this method returns false, the message will not be printed.
The timestamp is in seconds granularity. */
public bool ShouldPrintMessage(int timestamp, string message) {
for(var i = 0; i < n; i++){
(var hts, var hset) = hist[i];
if(timestamp - hts < n && hset.Contains(message))
return false;
}
(var ts, var set) = hist[timestamp % n];
if(ts != timestamp)
hist[timestamp % n] = (timestamp, set = new HashSet<string>());
set.Add(message);
return true;
}
}
/**
* Your Logger object will be instantiated and called as such:
* Logger obj = new Logger();
* bool param_1 = obj.ShouldPrintMessage(timestamp,message);
*/<file_sep>public class Solution {
public int KConcatenationMaxSum(int[] arr, int k) {
var res = 0L;
var sum = 0L;
var prev = 0L;
var delta = 0L;
var baseSum = 0L;
for(int n = 0; n < 3; n++){
for(int i = 0; i < arr.Length; i++){
sum = Math.Max(sum+arr[i], arr[i]);
res = Math.Max(res, sum);
}
if(prev >= res)
return (int)(prev % (Math.Pow(10, 9) + 7));
if(n==0){
baseSum = res;
} else if(n == 1){
delta = res-prev;
} else if(n == 2){
return (int)(((k-1)*delta+baseSum) % (Math.Pow(10, 9) + 7));
}
prev = res;
}
return 0;
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public void Flatten(TreeNode root) {
FlattenInternal(root);
}
/*
1
2 5
3 4 6
1 - 2 - 3 - 4 -
*/
public TreeNode FlattenInternal(TreeNode node) { //1
if(node == null) // false
return node;
var left = node.left; // 2
var right = node.right; // 5
node.right = null;
node.left = null;
TreeNode lastSubtreeNode = node;
// visit left subtree
if(left != null){ // t
lastSubtreeNode.right = left;
lastSubtreeNode = FlattenInternal(left); // 4
}
// visit right
if(right != null){
// contunie left subtree or curent node
lastSubtreeNode.right = right;
lastSubtreeNode = FlattenInternal(right); // 4
}
return lastSubtreeNode; // 4
}
}<file_sep>public class Solution {
public IList<string> WordsAbbreviation(IList<string> dict) {
var map = new Dictionary<string, List<int>>();
var n = dict.Count;
var res = new string[n];
for(int i=0; i<n; i++){
var w = dict[i];
if(w.Length < 4){
res[i] = w;
continue;
}
var key = Shorter(1, w);
if(!map.ContainsKey(key))
map[key] = new List<int>();
map[key].Add(i);
}
foreach(var kv in map){
if(kv.Value.Count == 1){
var idx = kv.Value[0];
res[idx] = kv.Key;
continue;
}
var trie = new Trie();
foreach(var idx in kv.Value){
var w = dict[idx];
var curr = trie;
for(var i = 1; i < w.Length; i++){
var c = w[i]-'a';
curr.Count++;
curr = curr.Children[c] ?? (curr.Children[c] = new Trie());
}
}
foreach(var idx in kv.Value){
var w = dict[idx];
var curr = trie;
var i = 1;
for(i = 1; i < w.Length && curr.Count > 1; i++)
curr = curr.Children[w[i]-'a'];
res[idx] = Shorter(i, w);
}
}
return res;
}
private string Shorter(int count, string str){
if(count > str.Length-3)
return str;
return str.Substring(0, count) + (str.Length-count-1).ToString() + str[str.Length-1];
}
public class Trie{
public Trie[] Children = new Trie[26];
public int Count;
}
}<file_sep>public class Solution {
public int StrStr(string haystack, string needle) {
if (needle == null || needle == string.Empty)
return 0;
var i = 0;
var n = 0;
while(n < needle.Length && i < haystack.Length){
if(haystack[i]==needle[n]){
n++;
i++;
}
else if(n > 0){
i -= (n-1);
n = 0;
} else{
i++;
}
}
return n == needle.Length ? i-n : -1;
}
}<file_sep>public class Solution {
public IList<string> AddOperators(string num, int target) {
var res = new List<string>();
void DFS(string eq, int start, long eval, long mult) {
if(start >= num.Length){
if(eval == target)
res.Add(eq);
return;
}
var n = 0L;
var end = num[start] == '0' ? start + 1 : num.Length;
for(int i = start; i < end; i++){
n = n*10 + num[i]-'0';
if(start == 0){
DFS(eq + n, i+1, n, n);
}
else{
DFS(eq + '+' + n, i+1, eval + n, n);
DFS(eq + '-' + n, i+1, eval - n, -n);
DFS(eq + '*' + n, i+1, eval - mult + mult * n, mult * n);
}
}
}
DFS("", 0 , 0, 0);
return res;
}
}<file_sep>public class Solution {
public int[] TopKFrequent(int[] nums, int k) {
var frq = new Dictionary<int, int>();
foreach(var n in nums)
if(frq.ContainsKey(n))
frq[n]++;
else
frq[n] = 1;
var arr = frq.Select(kv => new []{kv.Key, kv.Value}).ToArray();
void swap(int a, int b){
if(a == b)
return;
var t = arr[a];
arr[a] = arr[b];
arr[b] = t;
}
int partition(int lo, int hi){
var pivot = arr[hi];
for(int i = lo; i < hi; i++)
if(arr[i][1] < pivot[1])
swap(i, lo++);
swap(hi, lo);
return lo;
}
var lo = 0;
var hi = arr.Length - 1;
while(hi >= lo){
var mid = partition(lo, hi);
if(mid == k)
break;
if(mid > k)
hi = mid-1;
else
lo = mid+1;
}
var res = new int[k];
for(int i = 0; i < k; i++)
res[i] = arr[i][0];
return res;
}
}<file_sep>public class Solution {
public int MaxScore(int[] cardPoints, int k) {
var max = 0;
var cp = cardPoints;
var len = cp.Length;
var lo = len - k;
var hi = lo;
var sum = 0;
while(hi < len + k){
if(hi-lo >= k){
sum -= cp[lo % len];
lo++;
}
sum += cp[hi % len];
max = Math.Max(sum, max);
hi++;
}
return max;
}
}<file_sep>public class Solution {
public int Trap(int[] height) {
var n = height.Length;
if(n < 3)
return 0;
var lo = 0;
var hi = n-1;
var loMax = height[lo];
var hiMax = height[hi];
var res = 0;
while(lo < hi-1){
if(loMax <= hiMax){
var curr = height[++lo];
if(curr < loMax)
res += loMax - curr;
else
loMax = curr; // !!!
} else{
var curr = height[--hi];
if(curr < hiMax)
res += hiMax - curr;
else
hiMax = curr; // !!!
}
}
return res;
}
}<file_sep>public class Solution {
public int NumSubmat(int[][] mat) {
int rows = mat.Length, cols = mat[0].Length;
int res = 0;
for (int up = 0; up < rows; ++up) {
int[] h = Enumerable.Repeat(1, cols).ToArray();
for (int down = up; down < rows; ++down) {
for (int k = 0; k < cols; ++k)
h[k] &= mat[down][k];
var curr = countOneRow(h);
if(curr == 0)
break;
res += countOneRow(h);
}
}
return res;
}
private int countOneRow(int[] A) {
int res = 0, length = 0;
for (int i = 0; i < A.Length; ++i) {
length = (A[i] == 0 ? 0 : length + 1);
res += length;
}
return res;
}
}
<file_sep>public class MovingAverage {
LinkedList<int> data;
int size;
long sum;
/** Initialize your data structure here. */
public MovingAverage(int size) {
data = new LinkedList<int>();
this.size = size;
}
public double Next(int val) {
if(data.Count >= size){
var stale = data.First.Value;
data.RemoveFirst();
sum -= stale;
}
sum += val;
data.AddLast(val);
return sum/(double)data.Count;
}
}
/**
* Your MovingAverage object will be instantiated and called as such:
* MovingAverage obj = new MovingAverage(size);
* double param_1 = obj.Next(val);
*/<file_sep>public class Solution {
public IList<IList<string>> FindDuplicate(string[] paths) {
var map = new Dictionary<string, List<string>>();
foreach(var path in paths){
var infos = path.Split(" ");
if(infos.Length <= 1)
continue;
var dir = infos[0];
foreach(var file in infos.Skip(1)){
var fileInfo = file.Split("(");
var name = dir + "/" + fileInfo[0];
var content = fileInfo[1];
if(map.ContainsKey(content)){
map[content].Add(name);
} else{
map[content] = new List<string>{name};
}
}
}
return map
.Where(kv => kv.Value.Count > 1)
.Select(kv => (IList<string>)kv.Value)
.ToList();
}
}<file_sep>public class Solution {
public int[][] Multiply(int[][] A, int[][] B) {
var rows = A.Length;
var cols = A[0].Length;
var colsB = B[0].Length;
var res = new int[rows][];
// keep values of B != 0
// below it woulb the subject of the repetitive iterations
var helper = new List<(int, int)>[B.Length];
for(int r = 0; r < B.Length; r++){
helper[r] = new List<(int, int)>();
for(int c = 0; c < colsB; c++){
if(B[r][c] != 0)
helper[r].Add((c, B[r][c]));
}
}
for(int r = 0; r < rows; r++){
res[r] = new int[colsB];
for(int c = 0; c < cols; c++){
var a = A[r][c];
if(a != 0)
foreach(var (idx, val) in helper[c])
res[r][idx] += a * val;
}
}
return res;
}
}<file_sep>public class NumMatrix {
private int[,] m;
public NumMatrix(int[][] matrix) {
var rows = matrix.Length;
var cols = matrix[0].Length;
m = new int[rows, cols];
for(int i = 0; i < rows; i++)
for(int j = 0; j < cols; j++)
m[i,j] = matrix[i][j] + (j == 0 ? 0 : m[i, j-1]);
}
public int SumRegion(int row1, int col1, int row2, int col2) {
var sum = 0;
for(int r = row1; r <= row2; r++){
sum += m[r,col2] - (col1 == 0 ? 0 : m[r,col1-1]);
}
return sum;
}
}
/**
* Your NumMatrix object will be instantiated and called as such:
* NumMatrix obj = new NumMatrix(matrix);
* int param_1 = obj.SumRegion(row1,col1,row2,col2);
*/<file_sep>public class Solution {
public int MinSubArrayLen(int s, int[] nums) {
var lo = 0;
var hi = 0;
var sum = 0;
var res = int.MaxValue;
while(hi < nums.Length){
while(hi < nums.Length && sum < s){
sum += nums[hi++];
}
while(sum >= s && lo <= hi && lo < nums.Length){
res = Math.Min(res, hi - lo);
sum -= nums[lo++];
}
}
return res == int.MaxValue ? 0 : res;
}
}<file_sep>public class Solution {
public int FirstMissingPositive(int[] nums) {
int n = nums.Length;
// 1. mark numbers (num < 0) and (num > n) with a special marker number (n+1)
// (we can ignore those because if all number are > n then we'll simply return 1)
for (int i = 0; i < n; i++) {
if (nums[i] <= 0 || nums[i] > n) {
nums[i] = n + 1;
}
}
// note: all number in the array are now positive, and on the range 1..n+1
// 2. mark each cell appearing in the array, by converting the index for that number to negative
for (int i = 0; i < n; i++) {
int num = Math.Abs(nums[i]);
if (num > n) {
continue;
}
num--; // -1 for zero index based array (so the number 1 will be at pos 0)
if (nums[num] > 0) { // prevents double negative operations
nums[num] *= -1;
}
}
// 3. find the first cell which isn't negative (doesn't appear in the array)
for (int i = 0; i < n; i++) {
if (nums[i] >= 0) {
return i + 1;
}
}
// 4. no positive numbers were found, which means the array contains all numbers 1..n
return n + 1;
}
}<file_sep>public class Solution {
public int NumTrees(int n) {
if(n == 0)
return 0;
N = n;
return NumTrees(1, n);
}
private Dictionary<int, int> memo = new Dictionary<int, int>();
private int N = 0;
public int NumTrees(int start, int end) {
var count = 0;
if (start > end){
return 1;
}
var key = start*N+end;
if(memo.TryGetValue(key, out var res))
return res;
for(int i = start; i <= end; i++){
var lTrees = NumTrees(start, i-1);
var rTrees = NumTrees(i+1, end);
count += lTrees * rTrees;
}
memo[key] = count;
return count;
}
}<file_sep>public class Solution {
public string AlphabetBoardPath(string target) {
var sb = new List<char>();
var x = 0;
var y = 0;
foreach(var c in target){
var xt = (c - 'a') % 5;
var yt = (c - 'a') / 5;
while(y > yt){
sb.Add('U');
y--;
}
while(x > xt){
sb.Add('L');
x--;
}
while(x < xt){
sb.Add('R');
x++;
}
while(y < yt){
sb.Add('D');
y++;
}
sb.Add('!');
}
return new String(sb.ToArray());
}
}<file_sep>public class Solution {
public void Rotate(int[][] matrix) {
if (matrix == null || matrix.Length <= 1)
return;
var rank = matrix.Length;
for(var i=0; i < rank; i++){
for(var j=i; j < rank; j++){
var t = matrix[j][i];
matrix[j][i] = matrix[i][j];
matrix[i][j] = t;
}
}
for(var i=0; i < rank; i++){
for(var j=0; j < rank/2; j++){
var t = matrix[i][j];
matrix[i][j] = matrix[i][rank-j-1];
matrix[i][rank-j-1] = t;
}
}
}
}<file_sep>public class Solution {
public int MissingElement(int[] nums, int k) {
int missing(int idx) {
return nums[idx] - nums[0] - idx;
}
var n = nums.Length;
var diff = missing(n-1);
if(diff < k){
return nums[n-1]+k-diff;
}
var lo = 0;
var hi = n-1;
while(hi != lo){
var mid = lo + (hi-lo)/2;
diff = missing(mid);
if(diff < k){
lo = mid + 1;
} else {
hi = mid;
}
}
return nums[hi - 1] + k - missing(hi-1);
}
}<file_sep>public class Solution {
public int MinSumOfLengths(int[] arr, int target) {
var n = arr.Length;
// Optimization: it is not required to populate this array
var best = new int[n];
var bestSoFar = int.MaxValue;
var sum = 0;
var start = 0;
var res = int.MaxValue;
for(var i = 0; i < n; i++){
sum += arr[i];
while(sum > target && start < n){
sum -= arr[start++];
}
if(sum == target){
var currLen = i-start+1;
bestSoFar = Math.Min(bestSoFar, currLen);
if(start > 0 && best[start-1] != int.MaxValue)
res = Math.Min(res, best[start-1] + currLen);
// Optimization: minimum possible length is 2
if(res == 2)
return res;
}
best[i] = bestSoFar;
}
return res == int.MaxValue ? -1 : res;
}
}<file_sep>public class Solution {
public bool ValidateStackSequences(int[] pushed, int[] popped) {
var ptr = 0;
var n = pushed.Length;
var stack = new Stack<int>();
foreach(var pu in pushed){
stack.Push(pu);
while(stack.Any() && ptr < n && stack.Peek() == popped[ptr]){
stack.Pop();
ptr++;
}
}
return !stack.Any();
}
}<file_sep>public class RangeModule {
List<ValueTuple<int,int>> data;
public RangeModule() {
data = new List<ValueTuple<int,int>>();
// dirty hack for binary searxh simplification
data.Add((int.MinValue, int.MinValue));
data.Add((int.MaxValue, int.MaxValue));
}
public void AddRange(int left, int right) {
var next = FindNext(left);
while(next < data.Count){
(var start, var end) = data[next];
if(start > right)
break;
left = Math.Min(left, start);
right = Math.Max(right, end);
data.RemoveAt(next);
}
data.Insert(next, (left, right));
}
public bool QueryRange(int left, int right) {
(var start, var end) = data[FindNext(left)];
return start <= left && end >= right;
}
public void RemoveRange(int left, int right) {
var next = FindNext(left);
var temp = new List<ValueTuple<int,int>>();
while(next < data.Count){
(var start, var end) = data[next];
if(start > right)
break;
if(start < left)
temp.Add((start, left));
if(end > right)
temp.Add((right, end));
data.RemoveAt(next);
}
for(var i = temp.Count-1; i >= 0; i--)
data.Insert(next, temp[i]);
}
private int FindNext(int left){
var lo = 0;
var hi = data.Count-1;
while(lo < hi){
var mid = lo + (hi - lo)/2;
(_, var nextRight) = data[mid];
if(left == nextRight)
return mid;
if(left < nextRight)
hi = mid;
else
lo = mid+1;
}
return hi;
}
}
/**
* Your RangeModule object will be instantiated and called as such:
* RangeModule obj = new RangeModule();
* obj.AddRange(left,right);
* bool param_2 = obj.QueryRange(left,right);
* obj.RemoveRange(left,right);
*/<file_sep>public class Solution {
public int AssignBikes(int[][] workers, int[][] bikes) {
var memo = new Dictionary<ValueTuple<int,int>, int>();
int DFS(int w, int taken){
if(w == workers.Length)
return 0;
var key = (w,taken);
if(memo.ContainsKey(key))
return memo[key];
var min = int.MaxValue;
for(var b = 0; b < bikes.Length; b++){
if((taken & (1<<b)) > 0)
continue;
var dist = Math.Abs(workers[w][0]-bikes[b][0]) + Math.Abs(workers[w][1]-bikes[b][1]);
min = Math.Min(min, DFS(w+1, (taken | (1<<b)))+dist);
}
memo[key] = min;
return min;
}
return DFS(0, 0);
}
}<file_sep>public class Solution {
public int MinMeetingRooms(int[][] intervals) {
var n = intervals.Length;
if(n < 2)
return n;
Array.Sort(intervals, new CompareStart());
var rooms = new SortedSet<(int, int)>(new CompareEnd());
for(int i=0; i <n ; i++){
var meet = intervals[i];
if(rooms.Any()){
var room = rooms.First();
var isFree = room.Item1 <= meet[0];
//Console.WriteLine(room.Item1 + " " + meet[0] + " " + isFree);
if(isFree)
rooms.Remove(room);
}
rooms.Add((meet[1], i));
}
return rooms.Count;
}
class CompareStart : IComparer<int[]>{
public int Compare(int[] a, int[] b){
return a[0]-b[0];
}
}
class CompareEnd : IComparer<(int, int)>{
public int Compare((int, int) a, (int, int) b){
var dif = a.Item1 - b.Item1;
return dif != 0 ? dif : a.Item2-b.Item2;
}
}
}<file_sep>public class Solution {
public int ThirdMax(int[] nums) {
var dq = new int?[3];
for(int i = 0; i < nums.Length; i++){
var n = nums[i];
if((dq[2] == null || n > dq[2]) && dq[1] != n && dq[0] != n){
dq[2] = n;
var j = 2;
while(j > 0 && (dq[j-1] == null || dq[j] > dq[j-1])){
var t = dq[j];
dq[j] = dq[j-1];
dq[j-1] = t;
j--;
}
}
}
return dq[2] != null ? dq[2].Value : dq[0].Value;
}
}<file_sep>public class Solution {
public bool WordBreak(string s, IList<string> wordDict) {
if(wordDict?.Count == 0)
return false;
var n = s.Length;
var set = new HashSet<string>(wordDict);
var memo = new bool[n+1];
memo[0] = true;
for(int i = 1; i<= n; i++){
for(int j = i-1; j >=0; j--){
if(memo[j] && set.Contains(s.Substring(j, i-j))){
memo[i] = true;
break;
}
}
}
return memo[n];
}
public bool WordBreak(string s, IList<string> wordDict) {
if(wordDict?.Count == 0)
return false;
var n = s.Length;
var set = new HashSet<string>(wordDict);
var memo = new bool?[n];
bool CheckSubstring(int start){
if (start >= n)
return true;
if(memo[start] != null)
return memo[start].Value;
for (int end = start + 1; end <= n; end++) {
var str = s.Substring(start, end-start);
if(set.Contains(str) && CheckSubstring(end)){
memo[start] = true;
return true;
}
}
memo[start] = false;
return false;
}
return CheckSubstring(0);
}
}<file_sep>public class Solution {
public int CherryPickup(int[][] grid) {
var rows = grid.Length;
var cols = grid[0].Length;
var dp = new int[rows, cols, cols];
for(var r = rows-1; r >= 0; r--){
for(var c1 = 0; c1 < cols; c1++){
for(var c2 = 0; c2 < cols; c2++){
var val = c1 == c2 ? grid[r][c1] : grid[r][c1] + grid[r][c2];
var max = 0;
if(r != rows-1)
for(var i=c1-1; i<=c1+1; i++)
for(var j=c2-1; j<=c2+1; j++)
if(i >= 0 && j>=0 && i <cols && j< cols)
max = Math.Max(max, dp[r+1,i,j]);
dp[r,c1,c2] = val+max;
}
}
}
return dp[0,0,cols-1];
}
}<file_sep>public class Solution
{
public TreeNode BstFromPreorder(int[] preorder)
{
var index = 0;
return InsertValue(null, ref index, preorder);
}
public TreeNode InsertValue(int? max, ref int i, int[] arr)
{
if (i >= arr.Length || (max != null && arr[i] > max))
return null;
var node = new TreeNode(arr[i]);
i += 1;
node.left = InsertValue(arr[i-1], ref i, arr);
node.right = InsertValue(max, ref i, arr);
return node;
}
}<file_sep>public class RandomizedSet {
private Dictionary<int,int> map = new Dictionary<int, int>();
private List<int> list = new List<int>();
private Random rand = new Random();
/** Initialize your data structure here. */
public RandomizedSet() {
}
/** Inserts a value to the set. Returns true if the set did not already contain the specified element. */
public bool Insert(int val) {
if(map.ContainsKey(val))
return false;
list.Add(val);
map[val] = list.Count-1;
return true;
}
/** Removes a value from the set. Returns true if the set contained the specified element. */
public bool Remove(int val) {
if(!map.ContainsKey(val))
return false;
var idx = map[val];
if (list.Count-1 != idx){ // element is already at the end
list[idx] = list[list.Count-1];
map[list[idx]] = idx;
}
list.RemoveAt(list.Count-1);
map.Remove(val);
return true;
}
/** Get a random element from the set. */
public int GetRandom() {
var idx = rand.Next(0,list.Count);
return list[idx];
}
}
/**
* Your RandomizedSet object will be instantiated and called as such:
* RandomizedSet obj = new RandomizedSet();
* bool param_1 = obj.Insert(val);
* bool param_2 = obj.Remove(val);
* int param_3 = obj.GetRandom();
*/<file_sep>public class Solution {
private int[] prefixSums;
private int totalSum;
private Random rand;
public Solution(int[] w) {
prefixSums = new int[w.Length];
for (int i = 0; i < w.Length; ++i) {
totalSum += w[i];
prefixSums[i] = totalSum;
}
rand = new Random(totalSum);
}
public int PickIndex() {
double target = totalSum * rand.NextDouble();
var low = 0;
var high = prefixSums.Length;
while (low < high) {
int mid = low + (high - low) / 2;
if (target > prefixSums[mid])
low = mid + 1;
else
high = mid;
}
return low;
}
}
/**
* Your Solution object will be instantiated and called as such:
* Solution obj = new Solution(w);
* int param_1 = obj.PickIndex();
*/<file_sep>public class Solution {
public int FindCircleNum(int[][] isConnected) {
var rows = isConnected.Length;
if(rows == 0) return 0;
var cols = isConnected[0].Length;
var visited = new HashSet<int>();
var q = new Queue<int>();
var res = 0;
for(int r = 0; r < rows; r++){
if(visited.Contains(r))
continue;
visited.Add(r);
q.Enqueue(r);
while(q.Any()){
var city = q.Dequeue();
for(int c = 0; c < cols; c++){
if(isConnected[city][c] == 1 && !visited.Contains(c)){
visited.Add(c);
q.Enqueue(c);
}
}
}
res++;
}
return res;
}
}<file_sep>public class Solution {
public int MinReorder(int n, int[][] connections) {
var nei = new List<int>[n];
var res = 0;
for(int i = 0; i < n; i++)
nei[i] = new List<int>();
foreach(var con in connections){
nei[con[0]].Add(con[1]+n); // from city to next
nei[con[1]].Add(con[0]);
}
var q = new Queue<int>();
q.Enqueue(0);
var visited = new HashSet<int>();
visited.Add(0);
while(q.Any()){
var city = q.Dequeue();
foreach(var next in nei[city]){
var nextCity = next >= n ? next-n : next;
if(visited.Contains(nextCity))
continue;
if(next >= n)
res++;
q.Enqueue(nextCity);
visited.Add(nextCity);
}
}
return res;
}
}
public class Solution {
public int MinReorder(int n, int[][] connections) {
var nei = new List<int>[n];
foreach(var con in connections){
if(nei[con[0]] == null)
nei[con[0]] = new List<int>();
if(nei[con[1]] == null)
nei[con[1]] = new List<int>();
nei[con[0]].Add(con[1]+n);
nei[con[1]].Add(con[0]);
}
return FixDirection(0, nei, n, int.MinValue);
}
private int FixDirection(int city, List<int>[] nei, int max, int prevCity){
var res = 0;
for(int j = 0; j < nei[city].Count; j++){
var n = nei[city][j];
if(n == prevCity || n-max == prevCity)
continue;
if(n >= max){
n -= max;
res++;
}
res += FixDirection(n, nei, max, city);
}
return res;
}
}<file_sep>/**
* Definition for singly-linked list.
* public class ListNode {
* public int val;
* public ListNode next;
* public ListNode(int x) { val = x; }
* }
*/
public class Solution {
public bool IsPalindrome(ListNode head) {
if (head == null || head.next == null)
return true;
var slow = head;
var fast = head;
var history = new Stack<int>();
while(fast != null && fast.next != null){
history.Push(slow.val);
slow = slow.next;
fast = fast.next.next;
}
if (fast != null)
slow = slow.next;
while(history.Any()){
if (history.Pop() != slow.val)
return false;
slow = slow.next;
}
return true;
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
/*
0
1 2
3
*/
public IList<IList<int>> VerticalOrder(TreeNode root) {
var res = new List<IList<int>>();
if(root == null)
return res;
var map = new Dictionary<int, List<int>>(); // 0;03 -1;1 1;2
var q = new Queue<(TreeNode, int)>();
q.Enqueue((root, 0)); // 2;1
var max = 0;
var min = 0;
while(q.Any()){
var (node, level) = q.Dequeue(); // 3;0
if(node == null) //f
continue;
if(!map.ContainsKey(level)) // f
map[level] = new List<int>();
map[level].Add(node.val);
max = Math.Max(max, level); // 0
min = Math.Min(min, level); // -1
q.Enqueue((node.left, level-1));
q.Enqueue((node.right, level+1));
}
for(int i = min; i <=max; i++){
res.Add(map[i]);
}
return res;
}
}<file_sep>public class Solution {
public int DiameterOfBinaryTree(TreeNode root) {
int diameter = 0;
DiameterOfBinaryTree(root, ref diameter);
return diameter > 0 ? diameter-1 : 0;
}
public int DiameterOfBinaryTree(TreeNode node, ref int diameter) {
if(node == null)
return 0;
var diameterLeft = DiameterOfBinaryTree(node.left, ref diameter);
var diameterRight = DiameterOfBinaryTree(node.right, ref diameter);
diameter = Math.Max(diameter, diameterLeft+diameterRight+1);
return Math.Max(diameterLeft, diameterRight)+1;
}
}<file_sep>public class Solution {
public int MaxProfit(int[] inventory, int orders) {
var n = inventory.Length;
Array.Sort(inventory);
long res = 0;
long max = inventory[n-1];
for(int i = n-2; i >= -1; i--){
long cur = i != -1 ? inventory[i] : 0;
if(cur == max)
continue;
long available = n-1-i;
if(available * (max-cur) <= orders){
orders -= (int)(available * (max-cur));
res += ((max*max+max)/2 - (cur*cur+cur)/2)*available;
max = cur;
} else {
while(max > cur && orders > 0){
var count = Math.Min(available, orders);
orders -= (int)count;
res += max * count;
max--;
}
}
}
return (int)(res % 1000000007);
}
}<file_sep>public class Solution {
public int FindKthLargest(int[] nums, int k) {
var q = new Heap(k+1);
foreach(var n in nums){
q.Add(n);
if(q.len > k)
q.Pool();
}
return q.Peek();
}
}
public class Heap{
public int len;
int[] arr;
public Heap(int cap){
arr = new int[cap];
}
public int Peek(){
return arr[0];
}
public void Pool(){
arr[0] = arr[len-1];
len--;
HeapifyDown();
}
public void Add(int val){
arr[len] = val;
len++;
HeapifyUp();
}
int left(int idx) {return idx*2+1;}
int right(int idx) {return idx*2+2;}
int parent(int idx) {return (idx-1)/2;}
private void HeapifyDown(){
var idx = 0;
while(left(idx) < len){
var childIdx = left(idx);
if(right(idx) < len && arr[right(idx)] < arr[childIdx])
childIdx = right(idx);
if(arr[childIdx] > arr[idx])
break;
swap(idx, childIdx);
idx = childIdx;
}
}
private void HeapifyUp(){
var idx = len - 1;
while(idx > 0){
if(arr[parent(idx)] < arr[idx])
break;
swap(idx, parent(idx));
idx = parent(idx);
}
}
void swap(int a, int b){
var temp = arr[a];
arr[a] = arr[b];
arr[b] = temp;
}
}
<file_sep>public class BSTIterator {
private Stack<TreeNode> stack = new Stack<TreeNode>();
public BSTIterator(TreeNode root) {
TraverseLeft(root);
}
public int Next() {
node = stack.Pop();
TraverseLeft(node.right);
return node.val;
}
public bool HasNext() {
return stack.Any();
}
private void TraverseLeft(TreeNode node){
while(node != null){
stack.Push(node);
node = node.left;
}
}
}
<file_sep>public class Solution {
public int MinimumHammingDistance(int[] source, int[] target, int[][] allowedSwaps) {
var n = source.Length;
var dsu = new DSU(n);
foreach(var sw in allowedSwaps)
dsu.Union(sw[0], sw[1]);
var map = new Dictionary<int, List<int>>();
for(int i = 0; i < n; i++){
var p = dsu.Find(i);
if(!map.ContainsKey(p))
map[p] = new List<int>();
map[p].Add(i);
}
var res = 0;
foreach(var indexes in map.Values){
var targetMap = new Dictionary<int, int>();
foreach(var idx in indexes){
var val = target[idx];
if(!targetMap.ContainsKey(val))
targetMap[val] = 1;
else
targetMap[val]++;
}
foreach(var idx in indexes){
var val = source[idx];
if(targetMap.ContainsKey(val)){
targetMap[val]--;
if(targetMap[val] < 0)
res++;
}
else{
res++;
}
}
}
return res;
}
private class DSU{
private int[] arr;
public DSU(int n){
arr = Enumerable.Range(0, n).ToArray();
}
public void Union(int a, int b){
var pa = Find(a);
var pb = Find(b);
arr[pa] = pb;
}
public int Find(int a){
if(arr[a] == a)
return a;
return Find(arr[a]);
}
}
}<file_sep>public class Solution {
public int MaxArea(int h, int w, int[] horizontalCuts, int[] verticalCuts) {
var maxh = 0;
var maxv = 0;
horizontalCuts.Sort();
verticalCuts.Sort();
for(int i = 0; i <= horizontalCuts.Length; i++){
var prev = i == 0 ? 0 : horizontalCuts[i-1];
var next = i == horizontalCuts.Length ? h : horizontalCuts[i];
maxh = Math.Max(maxh, next-prev);
}
for(int i = 0; i <= verticalCuts.Length; i++){
var prev = i == 0 ? 0 : verticalCuts[i-1];
var next = i == verticalCuts.Length ? w : verticalCuts[i];
maxv = Math.Max(maxv, next-prev);
}
maxh = maxh == 0 ? h : maxh;
maxv = maxv == 0 ? w : maxv;
long res = (long)maxh * (long)maxv;
return (int)(res % 1000000007);
}
}<file_sep>public class Solution {
public void Rotate(int[] nums, int k) {
var n = nums.Length;
var count =0;
for(int start =0; count < n; start++){
var idx = start;
var memo = nums[idx];
do{
var pos= (idx+k)%n;
var t = nums[pos];
nums[pos] = memo;
memo = t;
idx = pos;
count++;
} while(idx != start);
}
}
}<file_sep>public class Solution {
public int CanCompleteCircuit(int[] gas, int[] cost) {
var n = gas.Length;
var total = 0;
var curr = 0;
var station = 0;
for(int i = 0; i < n; i++){
var balance = gas[i] - cost[i];
total += balance;
curr += balance;
if(curr < 0){
curr = 0;
station = i+1;
}
}
return total < 0 ? -1 : station;
}
}<file_sep>public class Solution {
public int LargestRectangleArea(int[] heights) {
var st = new Stack<int>();
st.Push(-1);
var len = heights.Length;
var max = 0;
for(int i = 0; i < len; i++){
var h = heights[i];
while(st.Peek() > -1 && heights[st.Peek()] > h){
var prevIdx = st.Pop();
var prevVal = heights[prevIdx];
var square = prevVal * (i - 1 - st.Peek());
max = Math.Max(max, square);
}
st.Push(i);
}
while(st.Peek() > -1){
var prevIdx = st.Pop();
var prevVal = heights[prevIdx];
var square = prevVal * (len - 1 - st.Peek());
max = Math.Max(max, square);
}
return max;
}
}<file_sep>public class Solution {
private int rows;
private int cols;
public int MaxAreaOfIsland(int[][] grid) {
rows = grid.Length;
cols = grid[0].Length;
var res = 0;
for(int i = 0; i < rows; i++){
for(int j = 0; j < cols; j++){
if(grid[i][j] == 1){
res = Math.Max(res, BFS(i, j, grid));
}
}
}
return res;
}
private int BFS(int row, int col, int[][] grid){
var res = 0;
var q = new Queue<ValueTuple<int, int>>();
q.Enqueue((row, col));
grid[row][col] = -1;
while(q.Any()){
(var r, var c) = q.Dequeue();
res++;
foreach((var rd, var cd) in Directions){
var nr = r+rd;
var nc = c+cd;
if(nr>=0 && nr < rows && nc >= 0 && nc < cols && grid[nr][nc] == 1){
q.Enqueue((nr, nc));
grid[nr][nc] = -1;
}
}
}
return res;
}
private ValueTuple<int,int>[] Directions = new []{(1,0),(-1,0),(0,1),(0,-1)};
}<file_sep>public class Solution {
public int TotalFruit(int[] tree) {
var lo = 0;
var hi = 0;
var max = int.MinValue;
var map = new Dictionary<int, int>();
while(hi<tree.Length){
map[tree[hi]] = hi;
if(map.Count == 3){
var minIdx = map.Min(kv => kv.Value);
map.Remove(map.First(kv => kv.Value == minIdx).Key);
lo = minIdx + 1;
} else if(hi-lo+1 > max){
max = hi - lo+1;
}
hi++;
}
return max;
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public IList<IList<int>> ZigzagLevelOrder(TreeNode root) {
var res = new List<IList<int>>();
if(root == null)
return res;
var prev = new Stack<TreeNode>();
prev.Push(root);
res.Add(new List<int>{root.val});
var forward = false;
while(prev.Any()){
var cur = new Stack<TreeNode>();
var level = new List<int>();
void AddNode(TreeNode n){
if(n != null){
level.Add(n.val);
cur.Push(n);
}
}
for(int count = prev.Count; count > 0; count--){
var node = prev.Pop();
if(forward){
AddNode(node.left);
AddNode(node.right);
} else {
AddNode(node.right);
AddNode(node.left);
}
}
prev = cur;
if(level.Any())
res.Add(level);
forward = !forward;
}
return res;
}
}<file_sep>public class Solution {
public bool IsAlienSorted(string[] words, string order) {
var comparer = new AlienComparer(order);
for(int i = 1; i < words.Length; i++){
if(comparer.Compare(words[i-1], words[i]) > 0)
return false;
}
return true;
}
public class AlienComparer: IComparer<string>{
private Dictionary<char, int> order;
public AlienComparer(string order){
this.order = new Dictionary<char, int>();
for(int i = 0; i < order.Length; i++)
this.order[order[i]] = i;
}
public int Compare(string a, string b){
var len = Math.Min(a.Length, b.Length);
for(int i = 0; i < len; i++){
var c1 = order[a[i]];
var c2 = order[b[i]];
if(c1 != c2)
return c1 - c2;
}
return a.Length-b.Length;
}
}
}<file_sep>public class Solution {
public int[] MaxSlidingWindow(int[] nums, int k) {
var res = new int[nums.Length-k+1];
var dq = new LinkedList<int>();
for(int i = 0; i < nums.Length; i++){
var n = nums[i];
// remove max if it out of window
if(dq.Any() && dq.First.Value == i-k)
dq.RemoveFirst();
// keep decreasing order of the dequeue
while(dq.Any() && nums[dq.Last.Value] <= n)
dq.RemoveLast();
dq.AddLast(i);
if(i-k+1 >= 0)
res[i-k+1] = nums[dq.First.Value];
}
return res;
}
}<file_sep>public class LRUCache {
private int cap;
private Dictionary<int, LinkedListNode<ValueTuple<int,int>>> map = new Dictionary<int, LinkedListNode<ValueTuple<int,int>>>();
private LinkedList<ValueTuple<int,int>> history = new LinkedList<ValueTuple<int,int>>();
public LRUCache(int capacity) {
cap = capacity;
}
public int Get(int key) {
if(map.TryGetValue(key, out var node)){
history.Remove(node);
history.AddLast(node);
return node.Value.Item2;
}
return -1;
}
public void Put(int key, int value) {
var containsKey = map.ContainsKey(key);
if(!containsKey && map.Count == cap){
var evicted = history.First;
history.Remove(evicted);
map.Remove(evicted.Value.Item1);
}
if(containsKey){
var node = map[key];
node.Value = (key, value);
history.Remove(node);
history.AddLast(node);
} else{
history.AddLast((key, value));
map[key] = history.Last;
}
}
}
/**
* Your LRUCache object will be instantiated and called as such:
* LRUCache obj = new LRUCache(capacity);
* int param_1 = obj.Get(key);
* obj.Put(key,value);
*/<file_sep>public class Solution {
public int MinTransfers(int[][] transactions) {
var balance = new Dictionary<int, int>();
foreach(var t in transactions){
if(!balance.ContainsKey(t[0]))
balance[t[0]] = 0;
if(!balance.ContainsKey(t[1]))
balance[t[1]] = 0;
balance[t[0]] += t[2];
balance[t[1]] -= t[2];
}
var d = balance.Values.Where(v => v != 0).ToArray();
var n = d.Length;
if(n == 0)
return 0;
int rebalance(int p){
while(p < n && d[p] == 0)
p++;
if(p == n)
return 0;
var count = int.MaxValue;
for(var i = p+1; i < n; i++){
if((d[p] > 0 && d[i] < 0) || (d[p] < 0 && d[i] > 0)){
d[i] += d[p];
count = Math.Min(count, rebalance(p+1)+1);
d[i] -= d[p];
}
}
return count;
}
return rebalance(0);
}
}<file_sep>public class SparseVector {
Dictionary<int,int> v = new Dictionary<int, int>();
public SparseVector(int[] nums) {
for(var i = 0; i < nums.Length; i++){
if(nums[i] != 0)
v[i] = nums[i];
}
}
// Return the dotProduct of two sparse vectors
public int DotProduct(SparseVector vec) {
if(vec.v.Count < v.Count)
return vec.DotProduct(this);
var res = 0;
foreach(var kv in v){
if(vec.v.TryGetValue(kv.Key, out var val))
res += val * kv.val;
}
return res;
}
}
// Your SparseVector object will be instantiated and called as such:
// SparseVector v1 = new SparseVector(nums1);
// SparseVector v2 = new SparseVector(nums2);
// int ans = v1.DotProduct(v2);<file_sep>/**
* Definition for singly-linked list.
* public class ListNode {
* public int val;
* public ListNode next;
* public ListNode(int val=0, ListNode next=null) {
* this.val = val;
* this.next = next;
* }
* }
*/
public class Solution {
public ListNode SwapPairs(ListNode head) {
ListNode prev = null;
ListNode res = null;
while(head != null && head.next != null){
var second = head.next;
var third = second.next;
second.next = head;
if(prev != null)
prev.next = second;
else
res = second;
prev = head;
head = third;
}
if (prev != null)
prev.next = head;
return res;
}
/*public ListNode SwapPairs(ListNode head) {
if(head == null || head.next == null)
return head;
var next = head.next;
var memo = next.next;
next.next = head;
head.next = SwapPairs(memo);
return next;
}*/
}<file_sep>public class Solution {
public string LongestPalindrome(string s) {
var n = s.Length;
var length = 1;
var start = 0;
for(var i = 1; i <= 2*n-1; i++){
var idx = i/2.0;
int lo = (int)idx;
int hi = idx == lo ? lo : lo+1;
while(lo >= 0 && hi < n && s[lo] == s[hi]){
var len = hi-lo+1;
if(len > length){
start = lo;
length = len;
}
lo--;
hi++;
}
}
return s.Substring(start, length);
}
}<file_sep>public class Solution {
public void NextPermutation(int[] nums) {
var n = nums.Length;
var idx = n - 2;
while(idx >= 0 && nums[idx] >= nums[idx+1])
idx--;
if(idx >= 0){
var idx2 = n-1;
while(nums[idx2] <= nums[idx])
idx2--;
var t = nums[idx];
nums[idx] = nums[idx2];
nums[idx2] = t;
}
idx++;
Array.Reverse(nums, idx, n-idx);
return;
}
}<file_sep>public class AutocompleteSystem {
TrieNode Trie;
TrieNode Current;
private StringBuilder sb;
public AutocompleteSystem(string[] sentences, int[] times) {
Trie = new TrieNode();
for(int i = 0; i < sentences.Length; i++){
AddSentence(sentences[i], times[i]);
}
Reset();
}
private void Reset(){
Current = Trie;
sb = new StringBuilder();
}
private int Idx(char c){
return c == ' ' ? 26 : c-'a';
}
private void AddSentence(string str, int frq){
var t = Trie;
t.Frq[str] = frq;
foreach(var c in str){
if(t.Children[Idx(c)] == null)
t.Children[Idx(c)] = new TrieNode();
t = t.Children[Idx(c)];
t.AddSentence(str, frq);
}
}
public IList<string> Input(char c) {
var res = new List<string>();
if(c == '#'){
var str = sb.ToString();
var frq = Trie.Frq.ContainsKey(str) ? Trie.Frq[str]+1 : 1;
AddSentence(str, frq);
Reset();
return res;
}
sb.Append(c);
if(Current == null || Current.Children[Idx(c)] == null){
Current = null;
return res;
}
Current = Current.Children[Idx(c)];
foreach(var bucket in Current.Hot){
foreach(var s in bucket.Value){
res.Add(s);
if(res.Count == 3)
return res;
}
}
return res;
}
class TrieNode{
public TrieNode[] Children = new TrieNode[27];
public Dictionary<string, int> Frq = new Dictionary<string, int>();
public SortedDictionary<int, SortedSet<string>> Hot = new SortedDictionary<int, SortedSet<string>>(new ReverseComparer());
public void AddSentence(string str, int frq){
// remove from prev bucket
if(Frq.ContainsKey(str)){
var bucket = Frq[str];
Hot[bucket].Remove(str);
}
Frq[str] = frq;
if(Hot.ContainsKey(frq))
Hot[frq].Add(str);
else
Hot[frq] = new SortedSet<string>{ str };
}
}
class ReverseComparer : IComparer<int>{
public int Compare(int a, int b){
return b-a;
}
}
}
/**
* Your AutocompleteSystem object will be instantiated and called as such:
* AutocompleteSystem obj = new AutocompleteSystem(sentences, times);
* IList<string> param_1 = obj.Input(c);
*/<file_sep>public class Solution {
public string ReorganizeString(string S) {
var n = S.Length;
var a = 26;
var frq = new int[a];
if(n < 2)
return S;
foreach(var c in S){
if (frq[c-'a'] == 0)
frq[c-'a'] = c-'a';
frq[c-'a'] += a;
}
Array.Sort(frq);
var ptr = 0;
var res = new char[n];
for(int i = a-1; i >= 0; i--){
var count = frq[i]/a;
var c = frq[i] % a;
if(count > (n+1)/2)
return "";
while(count-- > 0){
res[ptr] = (char)(c + 'a');
ptr = ptr < n-2 ? ptr+2 : 1;
}
}
return new String(res.ToArray());
}
}<file_sep>public class Solution {
public int UniquePaths(int m, int n) {
int Fact(int a){
return a == 0 ? 1 : a * Fact(a-1);
}
return Fact(n+m-2)/(Fact(n-1)*Fact(m-1)); // (n chose k combinations)
}
}<file_sep>public class Solution {
//
public int MaxSubArrayLen(int[] nums, int k) {
var map = new Dictionary<int, int>();
var max = 0;
var sum = 0;
for(int i = 0; i < nums.Length; i++){
sum += nums[i];
if(sum == k){
max = Math.Max(max, i+1);
} else if(map.ContainsKey(sum-k)){
max = Math.Max(max, i - map[sum-k]);
}
if(!map.ContainsKey(sum))
map[sum] = i;
}
return max;
}
}<file_sep>public class Solution {
public int MaximizeSweetness(int[] sweetness, int K) {
var n = sweetness.Length;
var lo = 0;
var hi = 0;
for(int i =0; i < n; i++){
lo = Math.Min(lo, sweetness[i]);
hi += sweetness[i];
}
while(lo < hi){
var mid = lo + (hi-lo)/2 + 1;
var cuts = 0;
var sum = 0;
for(int i = 0; i < n; i++){
sum += sweetness[i];
if(sum >= mid){
sum = 0;
if(++cuts == K+1)
break;
}
}
if(cuts < K+1)
hi = mid-1;
else
lo = mid;
}
return lo;
}
}<file_sep>public class Solution {
public IList<string> MostVisitedPattern(string[] username, int[] timestamp, string[] website) {
int len = username.Length;
string[] orderedSites = website.Distinct().OrderBy(x => x).ToArray();
int n = orderedSites.Length;
Dictionary<string, int> siteOrder = new Dictionary<string, int>();
for (int i = 0; i < n; i++) {
siteOrder[orderedSites[i]] = i;
}
Dictionary<string, List<Tuple<int, int>>> dict = new Dictionary<string, List<Tuple<int, int>>>();
for (int i = 0; i < len; i++) {
if (!dict.ContainsKey(username[i])) {
dict[username[i]] = new List<Tuple<int, int>>();
}
dict[username[i]].Add(new Tuple<int, int>(timestamp[i], siteOrder[website[i]]));
}
int[] tab = new int[n * n * n];
foreach (string key in dict.Keys) {
int[] temp = dict[key].OrderBy(x => x.Item1).Select(x => x.Item2).ToArray();
HashSet<int> visited = new HashSet<int>();
for (int i = 0; i < temp.Length; i++) {
for(int j = i + 1; j < temp.Length; j++) {
for(int k = j + 1; k < temp.Length; k++) {
int t = ((temp[i] * n) + temp[j]) * n + temp[k];
if (!visited.Contains(t)) {
tab[t]++;
visited.Add(t);
}
}
}
}
}
int max = 0;
int res = 0;
for (int i = 0; i < tab.Length; i++) {
if (tab[i] > max) {
res = i;
max = tab[i];
}
}
return new List<string> { orderedSites[res / (n * n)], orderedSites[(res % (n * n)) / n], orderedSites[res % n] };
}
}<file_sep>public class Solution {
public int MaxDistToClosest(int[] seats) {
var max = -1;
var firstOccupied = -1;
var lastOccupied = -1;
var i = 0;
while(i < seats.Length){
if(seats[i] == 1){
if (firstOccupied == -1)
firstOccupied = i;
lastOccupied = i;
}
var counter = 0;
while(i < seats.Length && seats[i] == 0){
counter++;
i++;
}
if(counter > 0 && counter > max)
max = counter;
if(counter == 0)
i++;
}
return Math.Max((int)Math.Floor((max+1)/2.0), Math.Max(firstOccupied, seats.Length-1-lastOccupied));
}
}<file_sep>public class Solution {
public int Rob(int[] nums) {
var len = nums.Length;
for(int i = 2; i < len; i++)
nums[i] += Math.Max(i > 2 ? nums[i-3] : 0, nums[i-2]); // choose from 2 prev maximums
return len < 2 ? nums[0] : Math.Max(nums[len-1], nums[len-2]);
}
}
public class Solution {
public int Rob(int[] nums) {
var len = nums.Length;
for(int i = 2; i < len; i++){
var max = Math.Max(nums[i-2], nums[i-1]);
for(int j = 0; j <= i-2; j++){
max = Math.Max(max, nums[j]);
}
nums[i] += max;
}
return len < 2 ? nums[0] : Math.Max(nums[len-1], nums[len-2]);
}
}<file_sep>public class Solution {
public int NumOfMinutes(int n, int headID, int[] manager, int[] informTime) {
for(int i = 0; i < n; i++)
if(informTime[i] > 0)
calcManagerialLine(i, manager, informTime);
return informTime.Max();
}
void calcManagerialLine(int id, int[] manager, int[] informTime){
if(manager[id] != -1){
calcManagerialLine(manager[id], manager, informTime);
informTime[id] += informTime[manager[id]];
manager[id] = -1;
}
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public int CountNodes(TreeNode root) {
if (root == null)
return 0;
var n = root;
var d = 0;
while(n.left != null){
n = n.left;
d++;
}
if (d == 0)
return 1;
var lo = 1;
var hi = (int)Math.Pow(2, d)-1;
while(lo <= hi){
var mid = lo + (hi-lo)/2;
if (Exists(root, mid, d))
lo = mid+1;
else
hi = mid -1;
}
return (int)Math.Pow(2, d) - 1 + lo;
}
public bool Exists(TreeNode n, int t, int d){
var lo = 0;
var hi = (int)Math.Pow(2, d)-1;
while(d > 0){
var mid = lo+(hi-lo)/2;
if (t>mid){
lo = mid +1;
n = n.right;
} else {
hi = mid-1;
n = n.left;
}
d--;
}
return n != null;
}
}<file_sep>public class Solution {
public void ReverseString(char[] s) {
var l = 0;
var r = s.Length-1;
while(r>l){
var t = s[l];
s[l] = s[r];
s[r] = t;
l++;
r--;
}
}
}<file_sep>public class Trie {
private Dictionary<char, Trie> Children = new Dictionary<char, Trie>();
private bool EndOfWord = false;
/** Initialize your data structure here. */
public Trie() {
}
/** Inserts a word into the trie. */
public void Insert(string word) {
if(!string.IsNullOrEmpty(word))
Insert(word.ToCharArray(), 0);
}
private void Insert(char[] word, int ptr){
if(ptr == word.Length){
EndOfWord = true;
return;
}
Trie child = null;
if(!Children.TryGetValue(word[ptr], out child)){
child = Children[word[ptr]] = new Trie();
}
child.Insert(word, ptr+1);
}
/** Returns if the word is in the trie. */
public bool Search(string word) {
if(!string.IsNullOrEmpty(word))
return Search(word.ToCharArray(), 0, true);
return false;
}
private bool Search(char[] word, int ptr, bool checkEnd) {
if(word.Length == ptr){
return checkEnd ? EndOfWord : true;
}
if(Children.TryGetValue(word[ptr], out var child)){
return child.Search(word, ptr+1, checkEnd);
}
return false;
}
/** Returns if there is any word in the trie that starts with the given prefix. */
public bool StartsWith(string prefix) {
if(!string.IsNullOrEmpty(prefix))
return Search(prefix.ToCharArray(), 0, false);
return false;
}
}
/**
* Your Trie object will be instantiated and called as such:
* Trie obj = new Trie();
* obj.Insert(word);
* bool param_2 = obj.Search(word);
* bool param_3 = obj.StartsWith(prefix);
*/<file_sep>public class Solution {
public int Jump(int[] nums) {
var n = nums.Length;
if(n <= 1)
return 0;
var curJump = nums[0];
var nextJump = curJump;
var res = 1;
for(int i = 1; i < n; i++){
if(curJump < i){
curJump = nextJump;
res++;
}
nextJump = Math.Max(nextJump, nums[i]+i);
}
return res;
}
}<file_sep>public class Solution {
public int LongestConsecutive(int[] nums) {
var num_set = new HashSet<int>(nums);
int longestStreak = 0;
foreach (int num in num_set) {
if (!num_set.Contains(num-1)) {
int currentNum = num;
int currentStreak = 1;
while (num_set.Contains(currentNum+1)) {
currentNum += 1;
currentStreak += 1;
}
longestStreak = Math.Max(longestStreak, currentStreak);
}
}
return longestStreak;
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public bool FlipEquiv(TreeNode root1, TreeNode root2) {
if(root1?.val != root2?.val){
return false;
}
if (root1 == null)
return true;
return FlipEquiv(root1.left, root2.right) && FlipEquiv(root1.right, root2.left) ||
FlipEquiv(root1.left, root2.left) && FlipEquiv(root1.right, root2.right);
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int x) { val = x; }
* }
*/
public class Solution {
public TreeNode LowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
bool DFS(TreeNode node){
if(node == null)
return false;
var l = DFS(node.left);
var r = DFS(node.right);
if(l && r || (node == p || node == q) && (r || l)){
root = node;
return false;
}
return node == p || node == q || r || l;
}
DFS(root);
return root;
}
}
/*
public class Solution {
public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
Map<TreeNode, TreeNode> parent = new HashMap<>();
Deque<TreeNode> stack = new ArrayDeque<>();
parent.put(root, null);
stack.push(root);
while (!parent.containsKey(p) || !parent.containsKey(q)) {
TreeNode node = stack.pop();
if (node.left != null) {
parent.put(node.left, node);
stack.push(node.left);
}
if (node.right != null) {
parent.put(node.right, node);
stack.push(node.right);
}
}
To find the lowest common ancestor, we need to find where is p and q and a way to track their ancestors.
A parent pointer for each node found is good for the job. After we found both p and q, we create a set of p's ancestors.
Then we travel through q's ancestors, the first one appears in p's is our answer.
Set<TreeNode> ancestors = new HashSet<>();
while (p != null) {
ancestors.add(p);
p = parent.get(p);
}
while (!ancestors.contains(q))
q = parent.get(q);
return q;
}
}
*/<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public TreeNode BuildTree(int[] preorder, int[] inorder) {
var preIdx = 0;
var n = preorder.Length-1;
var map = new Dictionary<int, int>();
for(int i = 0; i <= n; i++)
map[inorder[i]] = i;
TreeNode buildTree(int lo, int hi){
if(lo > hi)
return null;
var rootIdx = map[preorder[preIdx++]];
var root = new TreeNode(inorder[rootIdx]);
root.left = buildTree(lo, rootIdx-1);
root.right = buildTree(rootIdx+1, hi);
return root;
}
return buildTree(0, n);
}
}<file_sep>public class Solution {
public bool ValidPalindrome(string s) {
var l = 0;
var r = s.Length-1;
if(IsPalindrome(s, ref l, ref r))
return true;
var l2 = l+1;
var r2 = r-1;
return IsPalindrome(s, ref l, ref r2) || IsPalindrome(s, ref l2, ref r);
}
bool IsPalindrome(string s, ref int l, ref int r){
while(r >= l){
if(s[l] != s[r])
return false;
l++;
r--;
}
return true;
}
}<file_sep>public class Solution {
public int MaxProfit(int[] prices) {
int maxCurr = 0;
int maxSoFar = 0;
for(int i = 1; i < prices.Length; i++){
maxCurr += prices[i] - prices[i-1];
maxCurr = Math.Max(maxCurr, prices[i] - prices[i-1]);
maxSoFar = Math.Max(maxSoFar, maxCurr);
}
return maxSoFar;
}
}<file_sep>public class Solution {
public int ClosestValue(TreeNode root, double target) {
var res = root.val;
var delta = double.MaxValue;
while(root != null){
if((double)root.val == target)
return root.val;
var d = Math.Abs(root.val-target);
if(d < delta){
delta = d;
res = root.val;
}
root = (double)root.val > target ? root.left : root.right;
}
return res;
}
}<file_sep>public class Solution {
public int MyAtoi(string str) {
str = str.Trim();
if (str.Length == 0)
return 0;
var i = 0;
var sign = 1;
int res = 0;
int prev = 0;
if (str[0] == '-'){
i++;
sign = -1;
}
if (str[0] == '+'){
i++;
}
for(; i < str.Length; i++){
if (char.IsDigit(str[i])){
res *= 10;
res += (str[i]-'0');
if (sign == 1 && res < 0)
return int.MaxValue;
if (sign == -1 && res < 0)
return int.MinValue;
} else{
break;
}
}
return (int)res*sign;
}
}<file_sep>public class Solution {
public int MaximalRectangle(char[][] matrix) {
var rows = matrix.Length;
if(rows == 0)
return 0;
var cols = matrix[0].Length;
var hist = new int[cols];
var max = 0;
for(int r = 0; r < rows; r++){
for(int c = 0; c < cols; c++){
hist[c] = matrix[r][c] == '1' ? hist[c]+1 : 0;
}
max = Math.Max(max, LargestRectangleArea(hist));
}
return max;
}
public int LargestRectangleArea(int[] heights) {
var st = new Stack<int>();
st.Push(-1);
var len = heights.Length;
var max = 0;
for(int i = 0; i <= len; i++){
var h = i == len ? -1 : heights[i];
while(st.Peek() > -1 && heights[st.Peek()] > h){
var prevIdx = st.Pop();
var prevVal = heights[prevIdx];
var square = prevVal * (i - 1 - st.Peek());
max = Math.Max(max, square);
}
st.Push(i);
}
return max;
}
}<file_sep>public class Solution {
// ["az","ba"],
public IList<IList<string>> GroupStrings(string[] strings) {
var map = new Dictionary<int, Dictionary<string, List<string>>>(); // [2][az[az]]
foreach(var str in strings){ // ba
var len = str.Length; //2
if(!map.ContainsKey(len)){ // f
map[len] = new Dictionary<string, List<string>>();
map[len][str] = new List<string>{str};
continue;
}
if(len == 1){ // f
map[len].First().Value.Add(str);
continue;
}
var newGroup = true;
foreach(var group in map[len]){ // az[az ba]
if(IsShifted(group.Key, str)){ // t
group.Value.Add(str); //
newGroup = false;
break;
}
}
if(newGroup) // f
map[len][str] = new List<string>{str};
}
var res = new List<IList<string>>();
foreach(var d in map.Values){
foreach(var l in d.Values)
res.Add(l);
}
return res;
}
bool IsShifted(string a, string b){ // az ba
if(a[0] > b[0])
return IsShifted(b, a);
var shift = b[0] - a[0]; //1
var sb = new char[a.Length];
for(int i = 0; i < a.Length; i++){
var shifted = (a[i] - 'a' + shift) % 26 + 'a'; //
if(b[i] != (char)shifted)
return false;
}
return true;
}
}<file_sep>public class Solution {
public IList<int> DistanceK(TreeNode root, TreeNode target, int K) {
var st = new Stack<int>();
var res = new List<int>();
if(root == null || target == null)
return res;
if(K == 0){
res.Add(target.val);
return res;
}
var path = DFS(root, target);
if(path == null)
return res;
TreeNode prev = null;
while(path.Any() && K > 0){
var q = new Queue<TreeNode>();
q.Enqueue(path.Peek());
var d = K;
while(q.Any() && d > 0){
for(int i = q.Count; i > 0; i--){
var node = q.Dequeue();
if(node.left != null && node.left != prev)
q.Enqueue(node.left);
if(node.right != null && node.right != prev)
q.Enqueue(node.right);
}
d--;
}
while(q.Any())
res.Add(q.Dequeue().val);
K--;
prev = path.Dequeue();
if(K==0 && path.Any())
res.Add(path.Peek().val);
}
return res;
}
Queue<TreeNode> DFS(TreeNode node, TreeNode target){
if(node == null)
return null;
if(node.val == target.val){
var q = new Queue<TreeNode>();
q.Enqueue(node);
return q;
}
var path = DFS(node.left, target) ?? DFS(node.right, target);
if(path != null){
path.Enqueue(node);
return path;
}
return null;
}
}<file_sep>public class Solution {
public IList<IList<int>> GetSkyline(int[][] buildings) {
var n = buildings.Length;
var res = new List<IList<int>>();
var points = new int[n*2][];
var idx = 0;
foreach(var b in buildings){
points[idx++] = new int[]{b[0], -b[2]}; // x, h
points[idx++] = new int[]{b[1], b[2]}; // x, h
}
// sort by x if xa != xb
// greater hight goes first at start
// lesser hight goes frist at end
// start goes before end
Array.Sort(points, Comparer<int[]>.Create((a,b) => a[0] != b[0] ? a[0] - b[0] : a[1] - b[1]));
var map = new SortedDictionary<int, int>(Comparer<int>.Create((a,b) => b - a)); // sort by hight
map[0] = 0;
foreach(var p in points){
var h = p[1];
var maxHight = map.First().Key;
if(h < 0){ // start
h = -h; // !!!
map[h] = map.ContainsKey(h) ? map[h]+1 : 1;
} else { // end
map[h]--;
if(map[h] == 0)
map.Remove(h);
}
var newMax = map.First().Key;
if(maxHight != newMax)
res.Add(new List<int>{p[0], newMax});
}
return res;
}
}<file_sep>public class Solution {
private Trie Words;
private char[][] Board;
private HashSet<string> Result;
private int LenR;
private int LenC;
private int MaxLen = -1;
public IList<string> FindWords(char[][] board, string[] words) {
Result = new HashSet<string>();
if(board == null || board[0] == null || words == null || words.Length == 0)
return Result.ToList();
Board= board;
LenC = board[0].Length;
LenR = board.Length;
Words = new Trie();
foreach(var w in words){
Words.Insert(w);
if(w.Length > MaxLen)
MaxLen = w.Length;
}
for(var i = 0; i < LenR; i++)
for(var j =0; j < LenC; j++){
FindWords(i, j, "");
if(Result.Count == words.Length)
return Result.ToList();
}
return Result.ToList();
}
private void FindWords(int row, int col, string prefix){
var letter = Board[row][col];
if(letter == '#')
return;
prefix += letter.ToString();
if(prefix.Length > MaxLen || !Words.StartsWith(prefix))
return;
if(Words.Search(prefix))
Result.Add(prefix);
Board[row][col] = '#';
foreach((int r, int c) in GetAdjacent(row, col)){
FindWords(r,c, prefix);
}
Board[row][col] = letter;
}
private ValueTuple<int, int>[] Sides = new ValueTuple<int, int>[]{
(-1,0),
(1,0),
(0,-1),
(0,1)
};
private IEnumerable<ValueTuple<int, int>> GetAdjacent(int row, int col){
foreach((int r, int c) in Sides){
var nRow = row+r;
var nCol = col+c;
if (nRow >= 0 && nRow < LenR && nCol >=0 && nCol < LenC)
yield return (nRow, nCol);
}
}
public class Trie {
private Dictionary<char, Trie> Children = new Dictionary<char, Trie>();
private bool EndOfWord = false;
/** Initialize your data structure here. */
public Trie() {
}
/** Inserts a word into the trie. */
public void Insert(string word) {
if(!string.IsNullOrEmpty(word))
Insert(word.ToCharArray(), 0);
}
private void Insert(char[] word, int ptr){
if(ptr == word.Length){
EndOfWord = true;
return;
}
Trie child = null;
if(!Children.TryGetValue(word[ptr], out child)){
child = Children[word[ptr]] = new Trie();
}
child.Insert(word, ptr+1);
}
/** Returns if the word is in the trie. */
public bool Search(string word) {
if(!string.IsNullOrEmpty(word))
return Search(word.ToCharArray(), 0, true);
return false;
}
private bool Search(char[] word, int ptr, bool checkEnd) {
if(word.Length == ptr){
return checkEnd ? EndOfWord : true;
}
if(Children.TryGetValue(word[ptr], out var child)){
return child.Search(word, ptr+1, checkEnd);
}
return false;
}
/** Returns if there is any word in the trie that starts with the given prefix. */
public bool StartsWith(string prefix) {
if(!string.IsNullOrEmpty(prefix))
return Search(prefix.ToCharArray(), 0, false);
return false;
}
}
}<file_sep>/**
* Definition for singly-linked list.
* public class ListNode {
* public int val;
* public ListNode next;
* public ListNode(int val=0, ListNode next=null) {
* this.val = val;
* this.next = next;
* }
* }
*/
/**
* Definition for singly-linked list.
* public class ListNode {
* public int val;
* public ListNode next;
* public ListNode(int x) { val = x; }
* }
*/
public class Solution
{
public void ReorderList(ListNode head)
{
if (head == null)
{
return;
}
ReorderList(head.next, head);
}
private ListNode ReorderList(ListNode tail, ListNode parent)
{
if(tail == null)
return parent;
var head = ReorderList(tail.next, parent);
if(head == null || head == tail){
if(head != null)
head.next = null;
return null;
}
var next = head.next;
head.next = tail;
if(next == tail){
tail.next = null;
return null;
}
tail.next = next;
return next;
}
}
public class Solution {
public void ReorderList(ListNode head) {
var st = new Stack<ListNode>(); // 1 2
var node = head;
while(node != null){
st.Push(node);
node = node.next;
}
while(head != null){
var next = head.next;
var tail = st.Pop();
if(tail == head)
break;
head.next = tail;
head = head.next;
if(next == head)
break;
head.next = next;
head = head.next;
}
if(head != null)
head.next = null;
}
}<file_sep>public class Solution {
public int OpenLock(string[] deadends, string target) {
var dead = new HashSet<string>(deadends);
var visiteds = new HashSet<string>();
var visitede = new HashSet<string>();
if(dead.Contains("0000") || dead.Contains(target))
return -1;
if(target == "0000")
return 0;
var qs = new Queue<string>();
var qe = new Queue<string>();
qs.Enqueue("0000");
visiteds.Add("0000");
qe.Enqueue(target);
visitede.Add(target);
var level = 1;
while(qs.Any() && qe.Any()){
if(BFS(qs, visiteds, visitede, dead))
return level;
level++;
if(BFS(qe, visitede, visiteds, dead))
return level;
level++;
}
return -1;
}
bool BFS(Queue<string> q, HashSet<string> visited, HashSet<string> visitedOther, HashSet<string> deadSet){
for(var count = q.Count; count > 0; count--){
var node = q.Dequeue();
foreach(var nei in GetNeighbours(node)){
//Console.WriteLine(nei);
if (visitedOther.Contains(nei))
return true;
if(!visited.Contains(nei) && !deadSet.Contains(nei)){
visited.Add(nei);
q.Enqueue(nei);
}
}
}
return false;
}
IEnumerable<string> GetNeighbours(string str){
for(int i = 0; i < str.Length; i++){
var node = str.ToCharArray();
var c = (int)(node[i] - '0');
node[i] = (char)((c == 9 ? 0 : c+1) + '0');
yield return new String(node);
node[i] = (char)((c == 0 ? 9 : c-1) + '0');
yield return new String(node);
}
}
}<file_sep>public class Solution {
public bool IsValidSudoku(char[][] board) {
bool isValid(char c, int[] vals){
if(c == '.')
return true;
if(c < '1' || c > '9')
return false;
var idx = c - '1';
if(vals[idx] == 1)
return false;
vals[idx] = 1;
return true;
}
for(int i = 0; i < 9; i++){
var col = new int[9];
var row = new int[9];
for(int j = 0; j < 9; j++){
if (!isValid(board[i][j], row) || !isValid(board[j][i], col))
return false;
}
}
for(var k = 0; k < 9*3; k+=3){
var col = k % 9;
var row = (k / 9)*3;
var vals = new int[9];
for(int i = 0; i < 3; i++){
for(int j = 0; j < 3; j++){
if (!isValid(board[i + row][j + col], vals))
return false;
}
}
}
return true;
}
}<file_sep>public class Solution {
public bool CheckValidString(string s) {
int balance = 0;
foreach(var c in s){
if (c == ')')
balance--;
else
balance++;
if (balance < 0 )
return false;
}
if (balance == 0)
return true;
balance =0;
for(var i = s.Length -1; i >=0; i--){
if (s[i] == '(')
balance--;
else
balance++;
if (balance < 0)
return false;
}
return true;
}
}<file_sep>public class Solution {
public int ExpressiveWords(string S, string[] words) {
int count = 0;
foreach(String originalWord in words){
if(isStretchy(originalWord, S)){
count ++;
}
}
return count;
}
private bool isStretchy(String originalWord, String stretched){
int i = 0, j = 0;
while(i < originalWord.Length && j < stretched.Length){
//Check Rule 1
if(originalWord[i] != stretched[j]){
return false;
}
int repeated1 = getRepeatedLength(originalWord, i);
int repeated2 = getRepeatedLength(stretched, j);
//Check Rule 2
if((repeated1 >= repeated2 || repeated2 < 3) && repeated1 != repeated2){
return false;
}
i += repeated1;
j += repeated2;
}
//Check Rule 1
return i == originalWord.Length && j == stretched.Length;
}
private int getRepeatedLength(String word, int start){
int end = start;
while (end < word.Length && word[end] == word[start]){
end ++;
}
return end - start;
}
}<file_sep>public class Solution {
public IList<string> FindStrobogrammatic(int n) {
var addZero = !firstCall;
firstCall = false;
if(n == 0)
return new List<string>{""};
if(n == 1)
return new List<string>{"0","1","8"};
var prev = FindStrobogrammatic(n - 2);
var list = new List<string>();
foreach(var str in prev){
if(addZero)
list.Add($"0{str}0");
list.Add($"1{str}1");
list.Add($"6{str}9");
list.Add($"8{str}8");
list.Add($"9{str}6");
}
return list;
}
private bool firstCall = true;
}<file_sep>/**
* // This is the Master's API interface.
* // You should not implement it, or speculate about its implementation
* class Master {
* public int Guess(string word);
* }
*/
class Solution {
public void FindSecretWord(string[] wordlist, Master master) {
var attempts = 10;
var wl = wordlist;
var left = wl.Length;
while(attempts > 0 && left > 1){
var frq = new int[6,26];
foreach(var w in wl){
if(w == null)
continue;
for(int i = 0; i < 6; i++){
frq[i, w[i]-'a'] += 1;
}
}
string guess = null;
var best = -1;
foreach(var w in wl){
if(w == null)
continue;
var score = 0;
for(int i = 0; i < 6; i++){
score += frq[i, w[i]-'a'];
}
if(score > best){
guess = w;
best = score;
}
}
var match = master.Guess(guess);
for(var j = 0; j < wl.Length; j++){
var w = wl[j];
if(w == null)
continue;
var m = 0;
for(int i = 0; i < 6; i++){
if (w[i] == guess[i])
m++;
}
if(m != match)
wl[j] = null;
else
left++;
}
attempts--;
}
}
}<file_sep>/**
* Definition for a binary tree node.
* public class TreeNode {
* public int val;
* public TreeNode left;
* public TreeNode right;
* public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
public class Solution {
public IList<IList<int>> PathSum(TreeNode root, int targetSum) {
var res = new List<IList<int>>();
var r = PathSum(root, targetSum, 0);
if( r != null)
foreach(var st in r)
res.Add(st.ToList());
return res;
}
public List<Stack<int>> PathSum(TreeNode node, int targetSum, int sum) {
if(node == null)
return null;
if(node.left == null && node.right == null){
if(sum+node.val == targetSum){
var r = new List<Stack<int>>{new Stack<int>()};
r[0].Push(node.val);
return r;
}
return null;
}
var stl = PathSum(node.left, targetSum, sum+node.val);
var str = PathSum(node.right, targetSum, sum+node.val);
if(stl == null && str == null)
return null;
var l = stl ?? str;
if(str != null && stl != null)
foreach(var st in str)
l.Add(st);
foreach(var st in l)
st.Push(node.val);
return l;
}
}<file_sep>public class Solution {
public IList<IList<string>> AccountsMerge(IList<IList<string>> accounts) {
var dsu = new DSU();
var emailToName = new Dictionary<string, string>();
foreach(var acc in accounts){
var name = acc[0];
for(int i = 1; i < acc.Count; i++){
emailToName[acc[i]] = name;
dsu.Union(acc[1], acc[i]);
}
}
var emailGroups = new Dictionary<string, HashSet<string>>();
foreach(var email in emailToName.Keys){
var key = dsu.Find(email);
if(!emailGroups.ContainsKey(key))
emailGroups[key] = new HashSet<string>();
emailGroups[key].Add(email);
}
var res = new List<IList<string>>();
foreach(var kv in emailGroups){
var name = emailToName[kv.Key];
var acc = kv.Value.OrderBy(v => v, StringComparer.Ordinal).ToList();
acc.Insert(0, name);
res.Add(acc);
}
return res;
}
public class DSU{
public Dictionary<string, string> Nodes = new Dictionary<string, string>();
public void Union(string a, string b){
Nodes[Find(a)] = Find(b);
}
public string Find(string v){
if(!Nodes.ContainsKey(v))
Nodes[v] = v;
if(Nodes[v] != v)
Nodes[v] = Find(Nodes[v]);
return Nodes[v];
}
}
}<file_sep>public class Solution {
public bool IsBipartite(int[][] graph) {
var len = graph.Length;
var colors = new int[len];
for(int i = 0; i < len; i++){
if(colors[i] != 0)
continue;
var q = new Queue<int>();
q.Enqueue(i);
colors[i] = 1;
while(q.Any()){
var node = q.Dequeue();
var color = colors[node];
foreach(var nei in graph[node]){
if(colors[nei] == 0){
q.Enqueue(nei);
colors[nei] = color == 1 ? 2 : 1;
} else if(colors[nei] == color){
return false;
}
}
}
}
return true;
}
}<file_sep>public class Solution
{
public int MaxProfit(int k, int[] prices){
if (prices == null || prices.Length < 2 || k <= 0)
return 0;
if (prices.Length < 2 * k)
k = prices.Length/2;
return DFS(prices, 0, k, new int?[prices.Length, k+1]);
}
int DFS(int[] prices, int start, int k, int?[,] memo){
if(start > prices.Length-2)
return 0;
if (memo[start, k] != null)
return memo[start, k].Value;
var maxProfitTotal = int.MinValue;
var min = int.MaxValue;
for (int i = start; i < prices.Length; i++){
min = Math.Min(min, prices[i]);
var profit = prices[i]-min;
if (k > 1)
profit += DFS(prices, i + 1, k - 1, memo);
maxProfitTotal = Math.Max(maxProfitTotal, profit);
}
if(maxProfitTotal < 0)
maxProfitTotal = 0;
memo[start, k] = maxProfitTotal;
return maxProfitTotal;
}
}<file_sep>public class Codec {
// Encodes a tree to a single string.
public string serialize(TreeNode root) {
if(root == null)
return "";
var sb = new List<string>();
var q = new Queue<TreeNode>();
q.Enqueue(root);
while(q.Any()){
var node = q.Dequeue();
if(node != null){
sb.Add(node.val.ToString());
q.Enqueue(node.left);
q.Enqueue(node.right);
} else{
sb.Add("");
}
}
return string.Join(",", sb);
}
// Decodes your encoded data to tree.
public TreeNode deserialize(string data) {
if(data == "")
return null;
var treeValues = data.Split(",");
var n = treeValues.Length;
bool tryGetNode(int idx, out TreeNode node){
var hasValue = treeValues[idx] != "";
node = hasValue ? new TreeNode{val = int.Parse(treeValues[idx])} : null;
return hasValue;
}
tryGetNode(0, out var root);
var q = new Queue<TreeNode>();
q.Enqueue(root);
for(int i = 1; i < n; i++){
var node = q.Dequeue();
if(tryGetNode(i, out node.left))
q.Enqueue(node.left);
if(tryGetNode(++i, out node.right))
q.Enqueue(node.right);
}
return root;
}
}<file_sep>public class Solution {
public string DecodeString(string s) {
var ptr = 0;
var sb = new StringBuilder();
while(ptr < s.Length){
var c = s[ptr];
if(c > '0' && c <= '9')
sb.Append(Decode(s, ref ptr));
else
sb.Append(c);
ptr++;
}
return sb.ToString();
}
private string Decode(string s, ref int ptr){
var num = 0;
while(ptr < s.Length && s[ptr] != '['){
num *= 10;
num += (int)(s[ptr]-'0');
ptr++;
}
ptr++;
var sb = new StringBuilder();
while(ptr < s.Length && s[ptr] != ']'){
var c = s[ptr];
if(c > '0' && c <= '9')
sb.Append(Decode(s, ref ptr));
else{
sb.Append(c);
}
ptr++;
}
return string.Concat(Enumerable.Repeat(sb.ToString(), num));
}
}<file_sep>public class Solution {
public void Merge(int[] nums1, int m, int[] nums2, int n) {
var n1 = m-1;
var n2 = n-1;
var i = nums1.Length-1;
while(n1 >= 0 && n2 >= 0){
if(nums1[n1] > nums2[n2]){
nums1[i]=nums1[n1];
n1--;
} else{
nums1[i] = nums2[n2];
n2--;
}
i--;
}
while(n2 >= 0){
nums1[i] = nums2[n2];
n2--;
i--;
}
}
}<file_sep>/**
* The Read4 API is defined in the parent class Reader4.
* int Read4(char[] buf4);
*/
public class Solution : Reader4 {
/**
* @param buf Destination buffer
* @param n Number of characters to read
* @return The number of actual characters read
*/
// abcde
public int Read(char[] buf, int n) { // [] 5
var buf4 = new char[4];
var idx = 0; // 5
var len = 4; // 4
while(n > idx && len == 4){ // true
len = Read4(buf4); // 1
for(int i = 0; i < len && n > idx; i++) // 1
buf[idx++] = buf4[i];
}
return idx;
}
}<file_sep>public class Solution {
public int NumDecodings(string s) {
return DFS(s, 0, new int?[s.Length]);
}
public int DFS(string s, int start, int?[] memo){
if(start > s.Length-1)
return 1;
if(memo[start] != null)
return memo[start].Value;
var res = 0;
var num = s[start] - '0';
if(num > 0){
res += DFS(s, start+1, memo);
if(start+1 < s.Length){
num = num * 10 + s[start+1] - '0';
if(num <= 26)
res += DFS(s, start+2, memo);
}
}
memo[start] = res;
return res;
}
}<file_sep>public class Solution {
public bool SearchMatrix(int[][] matrix, int target) {
var rows = matrix.Length;
if(rows == 0)
return false;
var cols = matrix[0].Length;
var c = 0;
var r = rows-1;
while(r >=0 && c < cols){
var val = matrix[r][c];
if(target == val)
return true;
if(target > val)
c++;
else
r--;
}
return false;
}
}<file_sep>public class RLEIterator {
int[] a;
int reps = 0;
int ptr = -2;
public RLEIterator(int[] A) {
a = A;
}
public int Next(int n) {
while(reps < n && ptr + 2 < a.Length){
n -= reps;
ptr += 2;
reps = a[ptr];
}
if(reps < n){
reps = 0;
return -1;
}
reps -= n;
return a[ptr+1];
}
}
/**
* Your RLEIterator object will be instantiated and called as such:
* RLEIterator obj = new RLEIterator(A);
* int param_1 = obj.Next(n);
*/<file_sep>public class Solution {
public string AddBinary(string a, string b) {
var idxa = a.Length-1;
var idxb = b.Length-1;
var sb = new List<char>();
var carry = 0;
while(idxa >=0 || idxb >=0){
var sum = carry;
if(idxa >= 0)
sum += a[idxa--]-'0';
if(idxb >= 0)
sum += b[idxb--]-'0';
sb.Add((char)((sum % 2)+'0'));
carry = sum / 2;
}
if(carry == 1)
sb.Add((char)(carry+'0'));
sb.Reverse();
return new String(sb.ToArray());
}
}
<file_sep>class Solution {
public int CountSquares(int[][] matrix) {
var res = 0;
for(int i = 0; i < matrix.Length; i++)
for(int j = 0; j < matrix[0].Length; j++)
if(matrix[i][j] > 0){
if(i > 0 && j > 0)
matrix[i][j] = Math.Min(Math.Min(matrix[i-1][j], matrix[i][j-1]), matrix[i-1][j-1])+1;
res += matrix[i][j];
}
return res;
}
}<file_sep>public class Solution {
/*
1
*/
public Node TreeToDoublyList(Node root) { // 4
if(root == null)
return null;
var st = new Stack<Node>(); // 1
void TraverseLeft(Node n){
while(n != null){
st.Push(n);
n = n.left;
}
}
TraverseLeft(root);
var head = st.Peek(); // 1
Node node = null; // 1
while(st.Any()){
var n = st.Pop(); // 5
if(node == null){
node = n; // 1
} else {
node.right = n; // 1 - 2 - 3 - 4 -5
n.left = node;
node = n; // 5
}
if(n.right != null) // t
TraverseLeft(n.right);
}
node.right = head; // 1-1
head.left = node; // 1-1
return head;
}
}<file_sep>/**
* Definition for singly-linked list.
* public class ListNode {
* public int val;
* public ListNode next;
* public ListNode(int x) { val = x; }
* }
*/
public class Solution {
public ListNode MergeTwoLists(ListNode l1, ListNode l2) {
if(l1==null)
return l2;
if(l2==null)
return l1;
var result = new ListNode(0);
var l = result;
while(l1 != null && l2 != null)
{
if (l1.val < l2.val){
l.next = l1;
l1 = l1.next;
} else{
l.next = l2;
l2 = l2.next;
}
l = l.next;
}
var left = l1 != null ? l1 : l2;
while(left != null){
l.next = left;
left = left.next;
l = l.next;
}
return result.next;
}
}<file_sep>public class Solution {
public int[] AsteroidCollision(int[] a) {
var n = a.Length;
if(n < 2)
return a;
var res = new List<int>();
var st = new Stack<int>();
for(int i=0; i < n;){
var v = a[i];
if(v < 0){
if(!st.Any() || st.Peek() < -1*v){
if(st.Any())
st.Pop(); // positive destroyed
else
{
res.Add(v); // no collision
i++;
}
} else {
if(st.Peek() == -1*v) // both destroyed
st.Pop();
i++;
}
} else{
st.Push(v);
i++;
}
}
foreach(var v in st.Reverse())
res.Add(v);
return res.ToArray();
}
}<file_sep>public class Solution {
/*
1 2 2
0 -1 1
1 1 0
*/
public void WallsAndGates(int[][] rooms) {
var rows = rooms.Length;
var cols = rooms[0].Length;
var q = new Queue<(int,int)>();
for(int r = 0; r < rows; r++){
for(int c = 0; c < cols; c++){
if(rooms[r][c] == 0)
q.Enqueue((r,c)); // (0, 1) (2,2)
}
}
var distance = 1;
var directions = new (int, int)[]{(-1,0),(1,0),(0,1),(0,-1)};
while(q.Any()){
for(int i = q.Count; i > 0; i--){
var (row, col) = q.Dequeue();
foreach(var (deltaRow, deltaCol) in directions){
var r = row+deltaRow;
var c = col+deltaCol;
if(r >= 0 && r < rows && c >= 0 && c < cols && rooms[r][c] == int.MaxValue){
rooms[r][c] = distance;
q.Enqueue((r,c));
}
}
}
distance++;
}
}
}<file_sep>public class Solution {
public int LongestCommonSubsequence(string text1, string text2) {
if (text1.Length > text2.Length){
return LongestCommonSubsequence(text2, text1);
}
var l1 = text1.Length;
var l2 = text2.Length;
var memo = new int[l2+1,l1+1];
for(int i = 1; i <= l2; i++){
for(int j = 1; j <= l1; j++){
if (text2[i-1]==text1[j-1]){
memo[i,j] = memo[i-1,j-1]+1;
} else{
memo[i,j] = Math.Max(memo[i-1,j], memo[i,j-1]);
}
}
}
return memo[l2, l1];
}
}<file_sep>public class Solution {
public int LengthOfLIS(int[] nums) {
//return DFS(nums, 0, -1, new int?[nums.Length+1, nums.Length+1]);
var res = 0;
var len = nums.Length;
if(len < 2)
return len;
var dp = new int[len];
dp[0] = 1;
for(int i = 1; i < len; i++){
var max = 0;
for(int j = 0; j < i; j++){
if(nums[i] > nums[j])
max = Math.Max(max, dp[j]);
}
dp[i] = max+1;
res = Math.Max(max+1, res);
}
return res;
}
private int DFS(int[] nums, int cur, int prev, int?[,] memo){
if(cur == nums.Length)
return 0;
if(memo[cur, prev+1] != null)
return memo[cur, prev+1].Value;
var count = DFS(nums, cur+1, prev, memo);
var isIncreasing = prev == -1 || nums[cur] > nums[prev];
if(isIncreasing)
count = Math.Max(count, DFS(nums, cur+1, cur, memo)+1);
memo[cur, prev+1] = count;
return count;
}
}<file_sep>public class Solution {
public IList<int> SpiralOrder(int[][] matrix) {
var res = new List<int>();
var rows = matrix.Length-1;
if(rows == -1)
return res;
var cols = matrix[0].Length-1;
var frow = 0;
var fcol = 0;
while(fcol<=cols && frow<=rows){
for(int i = fcol; i <= cols; i++)
res.Add(matrix[frow][i]);
frow++;
for(int i = frow; i <= rows; i++)
res.Add(matrix[i][cols]);
cols--;
if(frow<=rows){
for(int i = cols; i >= fcol; i--)
res.Add(matrix[rows][i]);
rows--;
}
if(fcol<=cols){
for(int i = rows; i >= frow; i--)
res.Add(matrix[i][fcol]);
fcol++;
}
}
return res;
}
}<file_sep>public class Solution {
public void SortColors(int[] nums) {
var lo = 0;
var hi = nums.Length-1;
for(int i = 0; i < nums.Length; i++)
if(nums[i] == 0)
Swap(nums, lo++, i);
for(int i = nums.Length-1; i >= lo; i--)
if(nums[i] == 2)
Swap(nums, i, hi--);
}
private void Swap(int[] nums, int a, int b){
if(a == b)
return;
var t = nums[a];
nums[a] = nums[b];
nums[b] = t;
}
}<file_sep>public class Solution {
public IList<IList<int>> ThreeSum(int[] nums) {
Array.Sort(nums);
var res = new List<IList<int>>();
for(int i = 0; i < nums.Length && nums[i] <= 0; i++){
if (i == 0 || nums[i-1] != nums[i]){
TwoSum(nums, i, res);
}
}
return res;
}
public void TwoSum(int[] nums, int i, List<IList<int>> res) {
var lo = i+1;
var hi = nums.Length-1;
while(lo < hi){
var sum = nums[i] + nums[lo] + nums[hi];
if(sum > 0){
hi--;
} else if(sum < 0){
lo++;
} else{
res.Add(new List<int>{nums[i], nums[lo++], nums[hi--]});
while (lo < hi && nums[lo-1] == nums[lo]){
lo++;
}
}
}
}
}<file_sep>public class Solution {
public int[] ExclusiveTime(int n, IList<string> logs) {
var res = new Dictionary<int, int>();
var callStack = new Stack<Function>();
foreach(var entry in logs){
var f = new Function(entry);
if(f.Type == "start"){
callStack.Push(f);
} else {
var fStart = callStack.Pop();
var exclusive = f.Ts - fStart.Ts + 1;
if(!res.ContainsKey(f.Id))
res[f.Id] = 0;
res[f.Id] += exclusive-fStart.Inclusive;
if(callStack.Any())
callStack.Peek().Inclusive += exclusive;
}
}
var ans = new int[res.Count];
foreach(var kv in res)
ans[kv.Key] = kv.Value;
return ans;
}
class Function{
public int Ts;
public int Id;
public string Type;
public int Inclusive;
public Function(string entry){
var arr = entry.Split(":");
Id = int.Parse(arr[0]);
Type = arr[1];
Ts = int.Parse(arr[2]);
}
}
}<file_sep>public class Solution {
public bool IsPalindrome(string s) {
var l = 0;
var r = s.Length-1;
while (r >= l){
if(!char.IsLetterOrDigit(s[l])){
l++;
} else if(!char.IsLetterOrDigit(s[r])){
r--;
} else if(char.ToLower(s[l++]) != char.ToLower(s[r--]))
return false;
}
return true;
}
}<file_sep>public class Solution {
public double FindMedianSortedArrays(int[] nums1, int[] nums2) {
if(nums1.Length > nums2.Length)
return FindMedianSortedArrays(nums2, nums1);
var l1 = nums1.Length;
var l2 = nums2.Length;
var len = (l1+l2+1)/2;
var lo = 0;
var hi = l1;
while(lo <= hi){
var mid1 = lo + (hi-lo)/2;
var mid2 = len - mid1;
var vLeft1 = mid1 == 0 ? int.MinValue : nums1[mid1-1];
var vLeft2 = mid2 == 0 ? int.MinValue : nums2[mid2-1];
var vRight1 = mid1 == l1 ? int.MaxValue : nums1[mid1];
var vRight2 = mid2 == l2 ? int.MaxValue : nums2[mid2];
if(vLeft1 <= vRight2 && vLeft2 <= vRight1){
return (l1+l2) % 2 == 0
? (Math.Max(vLeft1, vLeft2) + Math.Min(vRight1, vRight2)) / 2.0
: Math.Max(vLeft1, vLeft2);
}
if(vLeft1 > vRight2)
hi = mid1-1;
else
lo = mid1+1;
}
return -1;
}
}<file_sep>public class Solution {
public int NumPairsDivisibleBy60(int[] time) {
var map = new Dictionary<int, int>();
foreach(var t in time){
var n = t%60;
if(map.ContainsKey(n))
map[n]++;
else
map[n] = 1;
}
var res = 0;
foreach(var term1 in map.Keys.ToArray()){
if(term1 == 30 || term1 == 0){
var n = map[term1]-1;
res += ((n*n+n)/2);
continue;
}
var term2 = 60-term1;
if(map.ContainsKey(term2)){
res += map[term1] * map[term2];
map[term1] = 0;
}
}
return res;
}
}<file_sep>public class Solution {
public int FindMaxLength(int[] nums) {
var equalSums = new Dictionary<int, int>();
equalSums[0] = -1;
int maxDistance = 0;
int prev = 0;
for(int i=0; i<nums.Length; i++){
var v = nums[i] == 0 ? -1 : 1;
var currentSum = prev + v;
prev = currentSum;
if (equalSums.TryGetValue(currentSum, out var firstIndexOccurance))
{
var distance = i-firstIndexOccurance;
if (distance > maxDistance)
maxDistance = distance;
}
else
{
equalSums[currentSum] = i;
}
}
return maxDistance;
}
}<file_sep>public class Solution {
public int ShortestDistance(int[][] grid) {
}
}<file_sep>public class Solution {
public bool IsNumber(string s) {
if(s == null || s.Length == 0)
return false;
var sign = new State{ Symbols = new HashSet<char>{'-', '+'}};
var digit = new State{ Symbols = new HashSet<char>{'0', '1', '2', '3', '4', '5', '6', '7', '8', '9'}};
var dot = new State{ Symbols = new HashSet<char>{'.'}};
sign.Next = new List<State>{dot, digit};
dot.Next = new List<State>{digit};
digit.Next = new List<State>{dot, digit};
var state = new State{Next = new List<State>{sign, digit, dot}};
var isExpVisited = false;
foreach(var c in s){
if(c == 'E' || c == 'e'){
if(isExpVisited || sign.Visited > 1 || dot.Visited > 1 || digit.Visited < 1)
return false;
// reset state machine
isExpVisited = true;
sign.Visited = digit.Visited = 0;
sign.Next = new List<State>{digit};
digit.Next = new List<State>{digit};
state = new State{Next = new List<State>{sign, digit}};
} else{
state = state.Next.FirstOrDefault(st => st.Symbols.Contains(c));
if(state == null)
return false;
state.Visited++;
}
}
return !(sign.Visited > 1 || dot.Visited > 1 || digit.Visited < 1);
}
class State{
public HashSet<char> Symbols;
public List<State> Next;
public int Visited;
}
}<file_sep>public class Solution {
public IList<IList<int>> Permute(int[] nums) {
var res = new List<IList<int>>();
var n = nums.Length;
void swap(int a, int b){
if(a != b){
var t = nums[a];
nums[a] = nums[b];
nums[b] = t;
}
}
void permute(int start){
if(start == n)
res.Add(new List<int>(nums)); // copy current !!!!
else
for(int i = start; i < n; i++){
swap(start, i);
permute(start+1);
swap(start, i);
}
}
permute(0);
return res;
}
}<file_sep>public class MyCalendar {
List<ValueTuple<int,int>> data;
public MyCalendar() {
data = new List<ValueTuple<int,int>>();
// dirty hack for binary searxh simplification
//data.Add((int.MinValue, int.MinValue));
// data.Add((int.MaxValue, int.MaxValue));
}
public bool Book(int start, int end) {
if(!data.Any()){
data.Add((start, end));
return true;
}
if(data.Count == 1){
(var iStart, var iEnd) = data[0];
//Console.WriteLine(iStart +" "+ end +" "+start +" "+ iEnd);
if(iStart > end || start < iEnd)
return false;
data.Insert(start < iStart ? 0 : 1, (start, end));
return true;
}
var lo = 0;
var hi = data.Count;
while(lo < hi){
var mid = lo + (hi - lo)/2;
(var next, _) = data[mid];
if(start == next)
return false;
if(start < next)
hi = mid;
else
lo = mid+1;
}
if (lo >= data.Count-1)
return data[data.Count-1].Item2 <= start;
if (lo == 0)
return end <= data[lo].Item1;
(var nextStart, _) = data[lo+1];
(_, var prevEnd) = data[lo];
if(nextStart < end || start < prevEnd)
return false;
data.Insert(lo, (start, end));
return true;
}
}
/**
* Your MyCalendar object will be instantiated and called as such:
* MyCalendar obj = new MyCalendar();
* bool param_1 = obj.Book(start,end);
*/<file_sep>public class AutocompleteSystem {
TrieNode Trie;
TrieNode Current;
private StringBuilder sb;
public AutocompleteSystem(string[] sentences, int[] times) {
Trie = new TrieNode();
for(int i = 0; i < sentences.Length; i++){
AddSentence(sentences[i], times[i]);
}
Reset();
}
private void Reset(){
Current = Trie;
sb = new StringBuilder();
}
private int Idx(char c){
return c == ' ' ? 26 : c-'a';
}
private void AddSentence(string str, int frq){
var trie = Trie;
foreach(var c in str){
if(trie.Children[Idx(c)] == null)
trie.Children[Idx(c)] = new TrieNode();
trie = trie.Children[Idx(c)];
}
trie.Sentence = str;
trie.Frq += frq;
}
public IList<string> Input(char c) {
var res = new List<string>();
if(c == '#'){
var str = sb.ToString();
AddSentence(str, 1);
Reset();
return res;
}
sb.Append(c);
if(Current == null || Current.Children[Idx(c)] == null){
Current = null;
return res;
}
Current = Current.Children[Idx(c)];
var heap = new Heap(3);
var q = new Queue<TrieNode>();
q.Enqueue(Current);
while(q.Any()){
var node = q.Dequeue();
if(node.Sentence != null)
heap.Add(node.Sentence, node.Frq);
foreach(var child in node.Children.Where(n => n != null))
q.Enqueue(child);
}
return heap.Take();
}
class TrieNode{
public TrieNode[] Children = new TrieNode[27];
public string Sentence;
public int Frq;
}
class Heap{
SortedDictionary<int, SortedSet<string>> map = new SortedDictionary<int, SortedSet<string>>();
int capacity;
public Heap(int cap){
capacity = cap;
}
public void Add(string str, int frq){
if(map.Count == capacity){
var smallest = map.First();
if(smallest.Key > frq)
return;
if(smallest.Key != frq)
map.Remove(smallest.Key);
}
if(map.ContainsKey(frq))
map[frq].Add(str);
else
map[frq] = new SortedSet<string>{str};
}
public List<string> Take(){
var top = new List<string>();
foreach(var bucket in map.Reverse()){
foreach(var s in bucket.Value){
top.Add(s);
if(top.Count == capacity)
return top;
}
}
return top;
}
}
}
<file_sep>public class Solution {
public bool CanJump(int[] nums) {
if(nums == null || nums.Length <= 1){
return true;
}
var lastGood = nums.Length - 1;
for(var pos = nums.Length - 2; pos >= 0; pos--){
if(pos+nums[pos] >= lastGood)
lastGood = pos;
}
return lastGood == 0;
/*var memo = new int[nums.Length];
memo[nums.Length-1] = 1;
for(var pos = nums.Length - 2; pos >= 0; pos--){
var jump = (int)Math.Min(nums[pos]+pos, nums.Length-1);
for(var i = pos + 1; i <= jump; i++){
if(memo[i] == 1){
memo[pos] = 1;
break;
}
}
}
return memo[0] == 1;*/
//return BackTrack(nums, 0, new int[nums.Length]);
}
/*private bool BackTrack(int[] nums, int pos, int[] memo){
if(pos == nums.Length-1 || memo[pos] == 1)
return true;
if(memo[pos] == -1)
return false;
var jump = (int)Math.Min(nums[pos]+pos, nums.Length-1);
for(var i = jump; i > pos; i--){
if(BackTrack(nums, i, memo)){
memo[pos] = 1;
return true;
}
}
memo[pos] = -1;
return false;
}*/
}<file_sep>public class Solution {
public IList<int> CountSmaller(int[] nums) {
var n = nums.Length;
var s = new List<int>(n);
var res = new int[n];
for(var i = n-1; i >= 0; i--){
var num = nums[i];
var last = s.Count-1;
if(last == -1 || s[0] >= num){
s.Insert(0, num);
res[i] = 0;
continue;
}
if(s[last] < num){
res[i] = s.Count;
s.Add(num);
continue;
}
var lo = 0;
var hi = last;
while(lo < hi){
var mid = lo + (hi-lo)/2;
if(s[mid] >= num)
hi = mid;
else
lo = mid + 1;
}
res[i] = lo;
s.Insert(lo, num);
}
return res;
}
}
// Binary index tree
public class Solution {
public List<Integer> countSmaller(int[] nums) {
List<Integer> res = new LinkedList<Integer>();
if (nums == null || nums.length == 0) {
return res;
}
// find min value and minus min by each elements, plus 1 to avoid 0 element
int min = Integer.MAX_VALUE;
int max = Integer.MIN_VALUE;
for (int i = 0; i < nums.length; i++) {
min = (nums[i] < min) ? nums[i]:min;
}
int[] nums2 = new int[nums.length];
for (int i = 0; i < nums.length; i++) {
nums2[i] = nums[i] - min + 1;
max = Math.max(nums2[i],max);
}
int[] tree = new int[max+1];
for (int i = nums2.length-1; i >= 0; i--) {
res.add(0,get(nums2[i]-1,tree));
update(nums2[i],tree);
}
return res;
}
private int get(int i, int[] tree) {
int num = 0;
while (i > 0) {
num +=tree[i];
i -= i&(-i);
}
return num;
}
private void update(int i, int[] tree) {
while (i < tree.length) {
tree[i] ++;
i += i & (-i);
}
}
}<file_sep>public class Solution {
public int DieSimulator(int n, int[] rollMax) {
var memo = new Dictionary<ValueTuple<int,int,int>, int>();
var m = (int)Math.Pow(10,9)+7;
int DFS(int roll, int prev, int len){
if(roll > n)
return 1;
var key = (roll, prev, len);
if(memo.ContainsKey(key))
return memo[key];
var res = 0;
for(int i = 1; i <=6; i++){
if(i == prev && rollMax[i-1] == len)
continue;
res = (res + DFS(roll+1, i, i == prev ? len+1 : 1)) % m;
}
memo[key] = res;
return res;
}
return DFS(1, -1, 0);
}
}<file_sep>/**
* The Read4 API is defined in the parent class Reader4.
* int Read4(char[] buf4);
*/
public class Solution : Reader4 {
/**
* @param buf Destination buffer
* @param n Number of characters to read
* @return The number of actual characters read
*/
private int buffPtr;
private int buffCnt;
private char[] buf4 = new char[4];
private bool eof;
public int Read(char[] buf, int n) {
if (n <= 0 || eof)
return 0;
var ptr = 0;
while(ptr < n){
if(buffCnt == 0){
buffPtr = 0;
buffCnt = Read4(buf4);
}
if(buffCnt == 0){
eof = true;
break;
}
while(buffCnt > 0 && ptr < n){
buf[ptr++] = buf4[buffPtr++];
buffCnt--;
}
}
return ptr;
}
}<file_sep>public class Solution {
public int Compress(char[] chars) {
var n = chars.Length;
var w = 0;
for(int r = 0; r < n; r++){
var start = r;
while(r < n-1 && chars[r+1] == chars[r])
r++;
chars[w++] = chars[r];
if(r > start)
foreach(var c in (r - start + 1).ToString())
chars[w++] = c;
}
return w;
}
} | f1551b518472b61b8d67de405b9e40c7d67cb5ad | [
"C#"
] | 290 | C# | Igorium/Leetcode | c390242ad7a487bca95f02918131978163376bdb | dd13c958a5593180b3aa9e597449f30aac402264 | |
refs/heads/master | <file_sep># Generated by Django 3.1 on 2020-08-09 07:29
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('activity', '0004_activitytimeline'),
]
operations = [
migrations.AlterField(
model_name='activity',
name='participant',
field=models.ManyToManyField(blank=True, to='activity.Student'),
),
]
<file_sep>from django.http import HttpResponse
from django.shortcuts import render, redirect
from rest_framework import viewsets
from .forms import ActivityForm
from .models import Activity
from .serializers import ActivitySerializer
class ActivityViewSet(viewsets.ModelViewSet) :
queryset = Activity.objects.all()
serializer_class = ActivitySerializer
# Create your views here.
def index(request):
editActivity = None
if 'editId' in request.GET:
editActivity = Activity.objects.get(id=request.GET['editId'])
if request.POST:
createForm = ActivityForm(request.POST, instance=editActivity)
if (createForm.is_valid()):
newAct = createForm.save(commit=False)
if 'isDelete' in request.POST :
if request.POST['isDelete']:
newAct.delete()
else :
newAct.save()
return redirect('/')
activities = Activity.objects.all()
if editActivity:
activityForm = ActivityForm(instance=editActivity)
else:
activityForm = ActivityForm()
return render(request, "activity/index.html", {
'title': "Hello From Title",
'activities': activities,
"activityForm": activityForm,
'editActivity': editActivity
})
<file_sep>from django import forms
from .models import Activity
class ActivityForm(forms.ModelForm) :
class Meta :
model = Activity
fields = ['activity_name',
'activity_type',
'activity_desc']<file_sep>from .models import Activity, ActivityTimeline, Student
from rest_framework import serializers
class ActivityTimelineSerializer(serializers.ModelSerializer):
class Meta:
model = ActivityTimeline
fields = ['id',
'activity_start',
'activity_stop',
'activity_plantitle']
class StudentSerializer(serializers.ModelSerializer):
class Meta:
model = Student
fields = ['id', 'code', 'name']
class ActivitySerializer(serializers.ModelSerializer):
participant = StudentSerializer(many=True,required=False)
activitytimeline_set = ActivityTimelineSerializer(many=True,required=False)
class Meta:
model = Activity
fields = ['id',
'activity_name',
'activity_type','activity_desc',
'activity_date','participant','activitytimeline_set']
<file_sep>from django.contrib import admin
from django.utils.html import format_html
from .models import Activity,Student,ActivityTimeline
# Register your models here.
class ActivityTimelineInline(admin.TabularInline) :
model = ActivityTimeline
class ActivityAdmin(admin.ModelAdmin) :
list_display = ['id','activity_name',
'act_type',
'activity_type',
'activity_date']
list_display_links = ['id','activity_name']
inlines = [
ActivityTimelineInline
]
COLORDICT = {
'AC' : 'red',
'CU' : 'green',
'SA' : 'blue',
}
save_as = True
fields = ('activity_name',
'activity_type',
'activity_desc',
'participant')
def act_type(self,obj):
return format_html(
'<span style="color : {}">{}</span>',
self.COLORDICT[obj.activity_type],
obj.activity_type
)
search_fields = ['activity_name']
pass
admin.site.register(Activity,ActivityAdmin)
admin.site.register(Student)
admin.site.register(ActivityTimeline)
<file_sep>from django.db import models
# Create your models here.
ACTIVITY_TYPES = [
('AC', 'ACADEMIC'),
('SA', 'STUDENT AFFAIR'),
('CU', 'CULTURAL'),
]
class Student(models.Model):
code = models.CharField(max_length=255)
name = models.CharField(max_length=255)
def __str__(self):
return f'{self.code} - {self.name}'
class Activity(models.Model):
class Meta:
verbose_name_plural = "Activities"
activity_name = models.CharField(max_length=255)
activity_type = models.CharField(max_length=2, choices=ACTIVITY_TYPES)
activity_desc = models.TextField(blank=True, null=True)
activity_date = models.DateTimeField(auto_now_add=True) # วันที่สร้างกิจกรรมขึ้นมาในระบบ
participant = models.ManyToManyField(Student,blank=True)
def __str__(self):
return f'{self.activity_name} - {self.activity_date}'
pass
class ActivityTimeline(models.Model):
activity = models.ForeignKey(Activity, on_delete=models.CASCADE)
activity_start = models.DateTimeField()
activity_stop = models.DateTimeField(blank=True, null=True)
activity_plantitle = models.CharField(max_length=255)
| 99d672d7169ac3ed3e00b9985299997557a62d5a | [
"Python"
] | 6 | Python | pchaow/djangoTeaching | aaafb8d74f938c7d32b5affe53ac219b36dc0298 | c39e45087dc92ff59cf04954ee993e1a2903f20c | |
refs/heads/master | <repo_name>billstclair/two-factor-authenticator<file_sep>/README.md
# Two-Factor Authenticator
`totp.html` is a stand-alone web page contaning JavaScript which generates a Time-based One-Time Password (TOTP) based on a user-entered secret key.
The page is live at [billstclair.com/two-factor-authenticator](https://billstclair.com/two-factor-authenticator/). It contains its own documentation.
Distilled and expanded from <NAME>'s [TOTP Debugger](https://github.com/google/google-authenticator/blob/master/libpam/totp.html) and released under the same Apache license. His file is in this repository as `totp-debugger.html`.
During development, I'll push the not-yet-ready-for-primetime page to [billstclair.github.io/two-factor-authenticator/totp.html](https://billstclair.github.io/two-factor-authenticator/totp.html).
<file_sep>/push-to-branch
#!/bin/bash
git checkout gh-pages
git merge master
git push
git checkout master
| f546d4d9225cf1db87162ca48b7437cdd0e884af | [
"Markdown",
"Shell"
] | 2 | Markdown | billstclair/two-factor-authenticator | 1947b7a599886d503a286ecd035d2bb89489aa7c | 269513280d3e3a246b0f3e10bc77ad2f0f3863ce | |
refs/heads/master | <file_sep><body class="teal lighten-4">
<?php
include('config/db_connect.php');
// write query for all detail
$sql = 'SELECT title, timeframe, id ,stat FROM details ORDER BY created_at';
// get the result set (set of rows)
$result = mysqli_query($conn, $sql);
// fetch the resulting rows as an array
$detail = mysqli_fetch_all($result, MYSQLI_ASSOC);
// free the $result from memory (good practise)
mysqli_free_result($result);
// close connection
mysqli_close($conn);
?>
<!DOCTYPE html>
<html>
<?php include('templates/header.php'); ?>
<h4 class="center grey-text">Pass requests for verification</h4>
<div class="container">
<div class="row">
<?php foreach($detail as $temp): ?>
<div class="col s6 m4">
<div class="card z-depth-0">
<img src="img/logo.png"class="temp">
<div class="card-content center">
<h6><?php echo "Purpose: " ;echo htmlspecialchars($temp['title']); ?></h6>
<h6 ><?php echo "Status: "; echo htmlspecialchars($temp['stat']); ?></h6>
<ul class="grey-text">
<?php echo "Time Slot " ; foreach(explode(',', $temp['timeframe']) as $ing): ?>
<li><?php echo htmlspecialchars($ing); ?></li>
<?php endforeach; ?>
</ul>
</div>
<div class="card-action right-align">
<a class="brand-text" href="poldet.php?id=<?php echo $temp['id'] ?>">more info</a>
</div>
</div>
</div>
<?php endforeach; ?>
</div>
</div>
<?php include('templates/footer.php'); ?>
</html><file_sep><?php
$name='Abhishek';
$age=30;
//echo $name;
?>
<!DOCTYPE html>
<html>
<body>
<div >
<?php echo $name; ?>
<?php echo $age; ?>
</div>
</body>
</html>
| 7dcfde9a2bb6a219af15df1a9a6e4b0ba71a75eb | [
"PHP"
] | 2 | PHP | abhishekkv/epass | a420b266fd5accd46c44450456130c9c5c39747d | 2e6d7a03dd25053e39c75b3eb4a202562397f803 | |
refs/heads/main | <repo_name>maweiche/Animates<file_sep>/public/javascript/login.js
// Get the modal
var loginModal = document.getElementById("loginModal");
var signupModal = document.getElementById("signupModal");
var isSignupActive =false;
var isLoginActive =false;
async function loginFormHandler(event) {
event.preventDefault();
const username = document.querySelector('#username-login').value.trim();
const password = document.querySelector('#password-login').value.trim();
if (username && password) {
const response = await fetch('/api/users/login', {
method: 'post',
body: JSON.stringify({
username,
password
}),
headers: { 'Content-Type': 'application/json' }
});
if (response.ok) {
document.location.replace('/');
} else {
alert('Failed to log in');
}
}
}
//login modal
function loginDisplay(event) {
event.preventDefault()
console.log("login modal display activated")
isLoginActive = true;
loginModal.style.display = "inline"
}
//signup modal
function signupDisplay(event) {
event.preventDefault()
console.log("signup modal activated");
isSignupActive = true;
signupModal.style.display = "inline"
}
// Hides login modal by clicking on window
// window.onclick = (function (event) {
// event.preventDefault();
// //console.log(event.target, loginModal, signupModal);
// if(isLoginActive){
// signupModal.style.display = "none"
// isLoginActive = false;
// console.log("deactivate login ", isLoginActive);
// }else {
// loginModal.style.display = "none"
// }
// if(isSignupActive){
// loginModal.style.display = "none"
// isSignupActive = false;
// console.log("deactivate signup ", isSignupActive);
// }else {
// signupModal.style.display = "none"
// }
// // if (event.target == signupModal) {
// // signupModal.style.display = "none"
// // }
// })
//click to redirect to signup modal
if (document.querySelector('#signupRedirect') !== null) {
document.querySelector('#signupRedirect').addEventListener('click', signupDisplay);
}
//Hides signup modal by clicking on window
// window.onclick = (function(event){
// if (event.target == signupModal){
// signupModal.style.display="none"
// }
// })
// console.log(document.querySelector('#login'));
if (document.querySelector('#login') !== null) {
document.querySelector('#login').addEventListener('click', loginDisplay);
}
// document.querySelector('#signup').addEventListener('click', signupDisplay);
document.querySelector('#submitLogin').addEventListener('click', loginFormHandler);<file_sep>/README.md
# Animates
For this project we used Bootstrap, CSS, Javascript, Two.js, and local storage on the front-end, and Node.js, Express.js, NPM, MySql, Sequelize ORM, Handlebars, JQuery, and GIF Encoder on the backend to create an interactive drawing application. We researched and utilized a variety of technologies (listed below) in order to make the app interactive, useful, and fun. We used a collaborative approach to the creation of this app by using pair programming. We maintained an organized task flow by utilizing Github's project cards, issue submissions and branch protection rules so that we could review and approve updates to the app.
## App Overview
This app allows users to log in or sign up using a username and login or by providing and email and creating a username and password. Once logged in, the user may direct themselves using the navigation panel on the left. From the navigation bar the user can access the explore page in which they can make animations.
## User Stories
<img src="/images/userstory1.png" alt="Screenshot of User Stories">
<img src="/images/userstory2.png" alt="Screenshot of User Stories">
<img src="/images/userstory3.png" alt="Screenshot of User Stories">
## Built With
* [Visual Studio Code](https://code.visualstudio.com/) - to create and modify the code on my local device
* [Bootstrap](https://getbootstrap.com/) - to structure html elements
* [CSS](https://developer.mozilla.org/en-US/docs/Web/CSS) - for styling of handlebars files
* [Javascript](https://www.javascript.com/) - for routing and functionality
* [jQuery](https://jquery.com/) - to add actions to the page
* [Two.js](https://two.js.org/) - to contruct an animation tool
* [Node.js](https://nodejs.org/en/) - to run our server
* [NPM](https://www.npmjs.com/) - to utilize packages
* [Express.js](https://expressjs.com/) - for web framework
* [MySql](https://www.mysql.com/) - for database access
* [Sequelize ORM](https://sequelize.org/) - For SQL server access
* [Handlebars](https://handlebarsjs.com/) - For design templating
* [GIF Encoder (svg2img)](https://www.npmjs.com/package/svg2img) - for in-memory conversion of images to svg
* [Git](https://git-scm.com/) - to track changes and push commits
* [GitHub](github.com) - to host the repository and deploy with GitHub pages
* [Heroku](Heroku.com) - to host the repository and deploy
## App Visual
<img src="images/create-screenshot.png" alt="Screenshot of Drawing Tool/Application"/>
<img src="images/main-screenshot.png" alt="Screenshot of Drawing Tool/Application"/>
## Deployed Link
https://peaceful-cliffs-69062.herokuapp.com/
## Future Developments
We plan to develop further a more extensive Explore page. We also plan to add more social media components such as a like feature and a friends list. A galleries feature will eventually have a polished interactive user interface.
## Repositories and Links
https://github.com/amackenzie26/Animates
https://peaceful-cliffs-69062.herokuapp.com/
https://amackenzie26.github.io/Animates/
## Authors
* <NAME> [https://www.linkedin.com/in/adamkruschwitz/]
* <NAME> [https://www.linkedin.com/in/mattweichel/]
* <NAME> [https://www.linkedin.com/in/alec-mackenzie-5119a5b8/]
<file_sep>/models/Animation.js
const { Model, DataTypes } = require('sequelize');
const sequelize = require('../config/connection');
class Animation extends Model {}
Animation.init(
{
id: {
type: DataTypes.INTEGER,
primaryKey: true,
autoIncrement: true,
allowNull: false
},
title: {
type: DataTypes.STRING(50),
defaultValue: 0,
allowNull: false
},
path: { // The path from the public directory to the
type: DataTypes.STRING(150),
allowNull: false
},
playbackSpeed: {
type: DataTypes.INTEGER,
allowNull: false,
},
author_id: {
type: DataTypes.INTEGER,
references: {
model: 'user',
key: 'id'
}
}
},
{
sequelize,
timestamps: false,
freezeTableName: true,
underscored: true,
modelName: 'animation'
});
module.exports = Animation;<file_sep>/config/connection.js
//import sequelize
const Sequelize = require('sequelize');
require('dotenv').config();
let sequelize;
//if deployed on heroku
if(process.env.JAWSDB_URL) {
sequelize = new Sequelize(process.env.JAWSDB_URL);
} else {
//localhost
sequelize = new Sequelize(process.env.DB_NAME, process.env.DB_USER, process.env.DB_PASSWORD, {
host: 'localhost',
dialect: 'mysql',
port: 3306
});
}
//export
module.exports = sequelize;<file_sep>/db/schema.sql
DROP DATABASE IF EXISTS animates_db;
CREATE DATABASE animates_db;<file_sep>/controllers/animations.js
const router = require('express').Router();
const { response } = require('express');
const { Animation } = require('../models');
router.get('/create', async (req, res) => {
try {
res.render('create-animation', {
loggedIn: req.session.loggedIn
});
} catch (err) {
console.log(err);
}
});
router.get('/:id', async (req, res) => {
try {
const animation = await Animation.findByPk(req.params.id);
const animationJSON = await animation.get({ plain: true });
res.render('animation', {
loggedIn: req.session.loggedIn,
animation: animationJSON
});
} catch (err) {
console.log(err);
}
});
module.exports = router;<file_sep>/public/javascript/drawing-tool.js
const drawSpace = $("#draw-space");
const linewidth = $("#linewidth");
const clearBtn = $("#clear");
const undoBtn = $("#undo");
const redoBtn = $("#redo");
const framesContainer = $("#frames");
const addFrameBtn = $("#add-frame");
const playBtn = $("#play");
const stopBtn = $("#stop");
const FPS = $("#fps");
const saveBtn = $("#save");
const loadBtn = $("#load");
const loadData = $("#data");
const title = $("#title");
let animation;
// Stack
let undoHistory = [];
const lastPosition = new Two.Anchor(0, 0);
// var two = new Two({
// width: drawSpace.width(),
// height: drawSpace.height()
// });
var frames = new LinkedList();
let curFrame = 0;
let line = null;
// console.log(drawSpace[0]);
addFrame();
// two.appendTo(drawSpace[0]); // Get's the DOM Element from the jquery object
// two.add(frames[0]);
function startDraw(event) {
// Set initial position
const x = event.clientX - $(event.target).closest('#draw-space').offset().left;
const y = event.clientY - $(event.target).closest('#draw-space').offset().top;
lastPosition.set(x, y);
// Add event listeners
drawSpace.mouseup(endDraw);
// drawSpace.mouseout(endDraw); // Doesn't work, triggers when going over another line for whatever reason.
drawSpace.mousemove(draw);
}
function draw(event) {
// Get the current position as a Two.Anchor
const x = event.clientX - $(event.target).closest('#draw-space').offset().left;
const y = event.clientY - $(event.target).closest('#draw-space').offset().top;
const curPosition = new Two.Anchor(x, y);
// If a line hasn't been created, start one. otherwise, add the new position to the line.
const frame = frames.getIndex(curFrame);
// console.log(frame);
if(!line) {
line = frame.makeCurve([lastPosition.clone(), curPosition.clone()], true); // Make sure to clone these so that the array has no shallow copies
line.noFill();
line.stroke = '#333';
line.linewidth = linewidth.val();
line.translation.clear();
// Adding an offset to account for a problem with drawing.
line.translation.set(-10, -35);
// For some reason two.js adds 2 vertices near 0,0 by default to the start of each curve. This removes those.
line.vertices.shift();
line.vertices.shift();
// Sets the end of each line to be a half circle.
line.cap = "round";
console.log(line);
// Add this line to the current frame
frame.add(line);
// Clear the undo history
undoHistory = [];
} else {
line.vertices.push(curPosition);
}
lastPosition.set(curPosition.x, curPosition.y);
frame.update();
}
function endDraw(event) {
// Remove event listeners
drawSpace.off('mouseup');
// drawSpace.off('mouseout');
drawSpace.off('mousemove');
// set the line to null so a new line is created on the next click
line = null;
}
function addFrame() {
// Create the new frame and add it to the scene
const frame = new Two({
width: drawSpace.width(),
height: drawSpace.height()
});
frame.appendTo(drawSpace[0]); // Get's the DOM Element from the jquery object
frame.add(new Two.Group());
curFrame = frames.size();
frames.push(frame);
console.log(frame);
// update display
presentFrame(frames.getIndex(curFrame));
// Add new frame button to the page
addButton(curFrame);
// TODO - add delete frame button
return frame;
}
function addButton(i) {
const button = $("<button>");
button.addClass("frame-button");
button.attr("data-frame", i);
framesContainer.append(button);
// add frame event listeners
button.on('click', (event) => {
const id = parseInt(event.target.dataset.frame, 10);
console.log(id);
curFrame = id;
presentFrame(frames.getIndex(id));
});
}
function presentFrame(frame) {
hideFrames();
frame.appendTo(drawSpace[0]); // Get's the DOM Element from the jquery object
frame.update();
}
function hideFrames(frame) {
drawSpace.empty();
}
drawSpace.on('mousedown', startDraw);
// Clear button
clearBtn.on('click', (event) => {
const confirmed = confirm("Are you sure you want to clear your frame?");
if(confirmed) {
const frame = frames.getIndex(curFrame)
// Remove all children from the current group
frame.clear();
// Clear undo history
undoHistory = [];
frame.update();
}
});
// Undo button
undoBtn.on('click', (event) => {
const frame = frames.getIndex(curFrame);
if(frame.scene.children.length > 1) {
const undid = frame.scene.children.pop();
// console.log(undid);
undoHistory.push(undid);
frame.update();
}
});
// Redo button
redoBtn.on('click', (event) => {
const frame = frames.getIndex(curFrame);
const redid = undoHistory.pop();
if(redid) {
frame.scene.children.push(redid);
frame.update();
}
});
// Add frame button
addFrameBtn.on('click', addFrame);
// Add animation loop
function startAnimation() {
console.log('starting animation...')
if(!animation) {
console.log('setting animation interval...');
const framerate = Math.floor(1000.0 / FPS.val());
animation = setInterval(() => {
curFrame += 1;
if(curFrame === frames.size()) curFrame = 0;
presentFrame(frames.getIndex(curFrame));
}, framerate);
}
}
function stopAnimation() {
clearInterval(animation);
animation = null;
}
// Set play and stop button controls
playBtn.on('click', startAnimation);
stopBtn.on('click', stopAnimation);
// Save Animation
async function save() {
// Get the rendered SVG from the DOM
const svgs = [];
frames.forEach((frame) => {
presentFrame(frame);
// drawSpace.children();
svgs.push(drawSpace.html());
});
// console.log(svgs.toString());
const response = await fetch('/api/animations', {
method: 'POST',
body: JSON.stringify({
"animationData": svgs.toString(),
"playbackSpeed": Math.floor(1000.0 / FPS.val()),
title: title.val(),
width: $("#draw-space").width(),
height: $("#draw-space").height()
}),
headers: { 'Content-Type': 'application/json' }
});
if(response.ok) {
// Should redirect to the post page
document.location.replace('/');
} else {
alert('an error occured!');
}
}
saveBtn.on('click', save);
async function load(animationData) {
const svgs = animationData.split(",");
// TODO: add xmlns='http://www.w3.org/2000/svg'
frames.clear();
drawSpace.empty();
framesContainer.empty();
curFrame=-1;
svgs.forEach(svg => {
const frame = addFrame();
frame.interpret($(svg)[0]);
});
}
loadBtn.on('click', (event) => {
load(loadData.val());
}); | 10c06b422d069fb5b90bce50ec6aea8771c7df59 | [
"JavaScript",
"SQL",
"Markdown"
] | 7 | JavaScript | maweiche/Animates | 3cdbb4e7b77b97efe523ea6b383de722d6e2a850 | 910786c8018057254eb38690b831f85f777ad444 | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
namespace agenda
{
public class Program
{
public static void Main(String[] args)
{
ConectionMYSQL baseDeDatos = new ConectionMYSQL();
bool programaActivo = true;
do
{
Console.WriteLine("Selecciona lo que deseas hacer: \n1 - Mostrar agenda\n2 - Registrar nuevo contacto\n3 - Eliminar contacto\n4 - Editar contacto\n5 - Salir del programa");
int opcionSeleccionada = int.Parse(Console.ReadLine());
switch (opcionSeleccionada)
{
case 1:
List<Contacto> listaDeContactos = baseDeDatos.ObtenerContactos();
foreach (Contacto contacto in listaDeContactos)
{
Console.WriteLine("Nombre: " + contacto.Nombre);
Console.WriteLine("Correo electronico: " + contacto.Correo);
Console.WriteLine("Numero de telefono: " + contacto.Telefono);
Console.WriteLine("=============================");
}
break;
case 2:
Contacto nuevoContacto = new Contacto();
Console.WriteLine("\nIngresa el nombre de tu nuevo contacto");
nuevoContacto.Nombre = Console.ReadLine();
Console.WriteLine("\nIngresa el correo de tu nuevo contacto");
nuevoContacto.Correo = Console.ReadLine();
Console.WriteLine("\nIngresa el telefono de tu nuevo contacto");
nuevoContacto.Telefono = Console.ReadLine();
if (baseDeDatos.RegistrarContacto(nuevoContacto))
{
Console.WriteLine("\n===Registro exitoso===\n");
}else{
Console.WriteLine("Ocurrio un error durante el registro, intente de nuevo");
}
break;
case 3:
Console.WriteLine("\nIngresa el nombre del contacto a eliminar");
string nombreDeContacto = Console.ReadLine();
if (baseDeDatos.EliminarContacto(nombreDeContacto))
{
Console.WriteLine("\n===Eliminación exitosa===");
}else{
Console.WriteLine("\n===Ocurrio un error durante la eliminación===");
}
break;
case 4:
List<Contacto> contactos = baseDeDatos.ObtenerContactos();
foreach (Contacto contacto in contactos)
{
Console.WriteLine("- " + contacto.Nombre);
}
Console.WriteLine("Escribe el nombre del contacto a editar");
string nombreContacto = Console.ReadLine();
Console.WriteLine("¿Qué información deseas actualizar?\n1 - Correo\n2 - Telefono");
int infoAActualizar = int.Parse(Console.ReadLine());
switch (infoAActualizar)
{
case 1:
Console.WriteLine("\n===Ingresa el nuevo correo===\n");
string nuevoCorreo = Console.ReadLine();
if (baseDeDatos.ActualizarCorreo(nombreContacto,nuevoCorreo))
{
Console.WriteLine("\n===Aactualización exitosa===\n");
}else{
Console.WriteLine("\n===Ocurrio un error durante la actualización===\n");
}
break;
case 2:
Console.WriteLine("\n===Ingresa el nuevo telefono===\n");
string nuevoTelefono = Console.ReadLine();
if (baseDeDatos.ActualizarTelefono(nombreContacto, nuevoTelefono))
{
Console.WriteLine("\n===Aactualización exitosa===\n");
}else{
Console.WriteLine("\n===Ocurrio un error durante la actualización===\n");
}
break;
default:
Console.WriteLine("\n===Selecciona una opcion valida===\n");
break;
}
break;
case 5:
programaActivo = false;
break;
default:
Console.WriteLine("\n===Selecciona una opcion valida===\n");
break;
}
} while(programaActivo);
}
}
}<file_sep>namespace agenda
{
public class Contacto
{
public string Nombre { get; set; }
public string Correo { get; set; }
public string Telefono { get; set; }
}
}<file_sep>version: '3.9'
services:
bd-agenda:
image: mysql/mysql-server:latest
container_name: contenedor-bd
ports:
- "8080:3306"
restart: always
volumes:
- volumen-agenda:/var/lib/mysql
- ./bd/agenda.sql:/docker-entrypoint-initdb.d/agenda.sql:ro
command: ['mysqld', '--character-set-server=utf8mb4', '--collation-server=utf8mb4_0900_ai_ci']
environment:
MYSQL_ROOT_PASSWORD: "root"
MYSQL_HOST: localhost
MYSQL_DATABASE: "Agenda"
MYSQL_USER: "root"
app-agenda:
image: app-agenda
container_name: contenedor-agenda
build:
context: ./agenda/
dockerfile: Dockerfile
stdin_open: true
tty: true
depends_on:
- "bd-agenda"
environment:
DB_CONNECTION_STRING: "server=bd-agenda;user=admin;password=<PASSWORD>;database=Agenda"
volumes:
volumen-agenda:<file_sep>FROM mcr.microsoft.com/dotnet/aspnet:5.0-buster-slim AS base
WORKDIR /app
EXPOSE 80
EXPOSE 443
FROM mcr.microsoft.com/dotnet/sdk:5.0-buster-slim AS build
WORKDIR /src
COPY ["agenda.csproj", "./"]
RUN dotnet restore "agenda.csproj"
COPY . .
WORKDIR "/src/"
RUN dotnet build "agenda.csproj" -c Release -o /app/build
FROM build AS publish
RUN dotnet publish "agenda.csproj" -c Release -o /app/publish
FROM base AS final
WORKDIR /app
COPY --from=publish /app/publish .
# ENTRYPOINT ["dotnet", "agenda.dll"]<file_sep>-- MySQL Script generated by MySQL Workbench
-- Thu Mar 4 14:13:37 2021
-- Model: New Model Version: 1.0
-- MySQL Workbench Forward Engineering
SET @OLD_UNIQUE_CHECKS=@@UNIQUE_CHECKS, UNIQUE_CHECKS=0;
SET @OLD_FOREIGN_KEY_CHECKS=@@FOREIGN_KEY_CHECKS, FOREIGN_KEY_CHECKS=0;
SET @OLD_SQL_MODE=@@SQL_MODE, SQL_MODE='ONLY_FULL_GROUP_BY,STRICT_TRANS_TABLES,NO_ZERO_IN_DATE,NO_ZERO_DATE,ERROR_FOR_DIVISION_BY_ZERO,NO_ENGINE_SUBSTITUTION';
-- -----------------------------------------------------
-- Schema Agenda
-- -----------------------------------------------------
-- -----------------------------------------------------
-- Schema Agenda
-- -----------------------------------------------------
CREATE SCHEMA IF NOT EXISTS `Agenda` DEFAULT CHARACTER SET utf8 ;
USE `Agenda` ;
-- -----------------------------------------------------
-- Table `Agenda`.`Persona`
-- -----------------------------------------------------
CREATE TABLE IF NOT EXISTS `Agenda`.`Persona` (
`idPersona` INT NOT NULL AUTO_INCREMENT,
`Nombre` VARCHAR(45) NULL,
PRIMARY KEY (`idPersona`))
ENGINE = InnoDB;
-- -----------------------------------------------------
-- Table `Agenda`.`Telefono`
-- -----------------------------------------------------
CREATE TABLE IF NOT EXISTS `Agenda`.`Telefono` (
`idTelefono` INT NOT NULL AUTO_INCREMENT,
`Numero` VARCHAR(45) NULL,
`Persona_idPersona` INT NOT NULL,
PRIMARY KEY (`idTelefono`),
INDEX `fk_Telefono_Persona1_idx` (`Persona_idPersona` ASC) VISIBLE,
CONSTRAINT `fk_Telefono_Persona1`
FOREIGN KEY (`Persona_idPersona`)
REFERENCES `Agenda`.`Persona` (`idPersona`)
ON DELETE NO ACTION
ON UPDATE NO ACTION)
ENGINE = InnoDB;
-- -----------------------------------------------------
-- Table `Agenda`.`Correo`
-- -----------------------------------------------------
CREATE TABLE IF NOT EXISTS `Agenda`.`Correo` (
`idCorreo` INT NOT NULL AUTO_INCREMENT,
`Correo` VARCHAR(45) NULL,
`Persona_idPersona` INT NOT NULL,
PRIMARY KEY (`idCorreo`),
INDEX `fk_Correo_Persona_idx` (`Persona_idPersona` ASC) VISIBLE,
CONSTRAINT `fk_Correo_Persona`
FOREIGN KEY (`Persona_idPersona`)
REFERENCES `Agenda`.`Persona` (`idPersona`)
ON DELETE NO ACTION
ON UPDATE NO ACTION)
ENGINE = InnoDB;
-- -----------------------------------------------------
-- Data for table `inventario`.`Persona`
-- -----------------------------------------------------
START TRANSACTION;
USE `Agenda`;
INSERT INTO `Agenda`.`Persona` (`nombre`) VALUES ('Angel');
INSERT INTO `Agenda`.`Persona` (`nombre`) VALUES ('Mario');
INSERT INTO `Agenda`.`Persona` (`nombre`) VALUES ('Carlos');
INSERT INTO `Agenda`.`Persona` (`nombre`) VALUES ('Sandra');
INSERT INTO `Agenda`.`Persona` (`nombre`) VALUES ('Laura');
INSERT INTO `Agenda`.`Persona` (`nombre`) VALUES ('Zaira');
COMMIT;
-- -----------------------------------------------------
-- Data for table `inventario`.`Telefono`
-- -----------------------------------------------------
START TRANSACTION;
USE `Agenda`;
INSERT INTO `Agenda`.`Telefono` (`numero`,`Persona_idPersona`) VALUES ('2281819155', '1');
INSERT INTO `Agenda`.`Telefono` (`numero`,`Persona_idPersona`) VALUES ('2281915584', '2');
INSERT INTO `Agenda`.`Telefono` (`numero`,`Persona_idPersona`) VALUES ('2281365978', '3');
INSERT INTO `Agenda`.`Telefono` (`numero`,`Persona_idPersona`) VALUES ('2282456785', '4');
INSERT INTO `Agenda`.`Telefono` (`numero`,`Persona_idPersona`) VALUES ('1258745668', '5');
INSERT INTO `Agenda`.`Telefono` (`numero`,`Persona_idPersona`) VALUES ('2286597845', '6');
COMMIT;
-- -----------------------------------------------------
-- Data for table `inventario`.`Correo`
-- -----------------------------------------------------
START TRANSACTION;
USE `Agenda`;
INSERT INTO `Agenda`.`Correo` (`Correo`,`Persona_idPersona`) VALUES ('<EMAIL>', '1');
INSERT INTO `Agenda`.`Correo` (`Correo`,`Persona_idPersona`) VALUES ('<EMAIL>', '2');
INSERT INTO `Agenda`.`Correo` (`Correo`,`Persona_idPersona`) VALUES ('<EMAIL>', '3');
INSERT INTO `Agenda`.`Correo` (`Correo`,`Persona_idPersona`) VALUES ('<EMAIL>', '4');
INSERT INTO `Agenda`.`Correo` (`Correo`,`Persona_idPersona`) VALUES ('<EMAIL>', '5');
INSERT INTO `Agenda`.`Correo` (`Correo`,`Persona_idPersona`) VALUES ('<EMAIL>', '6');
COMMIT;
-- SET SQL_MODE = '';
-- DROP USER IF EXISTS admin;
SET SQL_MODE='ONLY_FULL_GROUP_BY,STRICT_TRANS_TABLES,NO_ZERO_IN_DATE,NO_ZERO_DATE,ERROR_FOR_DIVISION_BY_ZERO,NO_ENGINE_SUBSTITUTION';
CREATE USER 'admin' IDENTIFIED BY 'admin';
GRANT ALL PRIVILEGES ON *.* TO 'admin'@'%' WITH GRANT OPTION;
SET SQL_MODE=@OLD_SQL_MODE;
SET FOREIGN_KEY_CHECKS=@OLD_FOREIGN_KEY_CHECKS;
SET UNIQUE_CHECKS=@OLD_UNIQUE_CHECKS;<file_sep>using System;
using System.Collections.Generic;
using System.Threading.Tasks;
using MySqlConnector;
namespace agenda
{
public class ConectionMYSQL
{
private String connectionString;
private MySqlConnection conexion;
private string ID_PERSONA_ERROR = "0";
public ConectionMYSQL(){
connectionString = Environment.GetEnvironmentVariable("DB_CONNECTION_STRING");
conexion = new MySqlConnection(connectionString);
}
public List<Contacto> ObtenerContactos(){
List<Contacto> listaDeContactos = new List<Contacto>();
try
{
conexion.Open();
var comando = new MySqlCommand("SELECT Nombre,Numero,Correo FROM Persona,Telefono,Correo WHERE Persona.idPersona = Telefono.Persona_idPersona AND Persona.idPersona = Correo.Persona_idPersona;", conexion);
var reader = comando.ExecuteReader();
while (reader.Read())
{
Contacto contactoObtenido = new Contacto();
contactoObtenido.Nombre = reader.GetString(0);
contactoObtenido.Telefono = reader.GetString(1);
contactoObtenido.Correo = reader.GetString(2);
listaDeContactos.Add(contactoObtenido);
}
}
catch (Exception error)
{
System.Console.WriteLine("Ocurrio un error: " + error.Message);
}finally{
conexion.Close();
}
return listaDeContactos;
}
public bool RegistrarContacto(Contacto nuevoContacto){
bool registroExitoso = false;
if (RegistrarNombre(nuevoContacto.Nombre))
{
string idPersona = ObtenerId(nuevoContacto.Nombre);
if (RegistrarCorreo(nuevoContacto.Correo, idPersona) && RegistrarTelefono(nuevoContacto.Telefono, idPersona))
{
registroExitoso = true;
}
}
return registroExitoso;
}
public bool EliminarContacto(string nombreContacto){
bool eliminacionExitosa = false;
string idContacto = ObtenerId(nombreContacto);
if(EliminarTelefono(idContacto) && EliminarCorreo(idContacto) && EliminarNombre(idContacto)){
eliminacionExitosa = true;
}
return eliminacionExitosa;
}
public bool ActualizarCorreo(string nombreContacto, string nuevoCorreo){
bool actualizacionExitosa = false;
string idPersona = ObtenerId(nombreContacto);
try
{
conexion.Open();
var registroPersona = new MySqlCommand($"UPDATE Correo SET Correo = '{nuevoCorreo}' WHERE Persona_idPersona='{idPersona}'", conexion);
registroPersona.ExecuteNonQuery();
actualizacionExitosa = true;
} catch(Exception error){
Console.WriteLine("Ocurrió un error (Método ActualizarCorreo): " + error.Message);
}finally{
conexion.Close();
}
return actualizacionExitosa;
}
public bool ActualizarTelefono(string nombreContacto, string nuevoTelefono){
bool actualizacionExitosa = false;
string idPersona = ObtenerId(nombreContacto);
try
{
conexion.Open();
var registroPersona = new MySqlCommand($"UPDATE Telefono SET Numero = '{nuevoTelefono}' WHERE Persona_idPersona='{idPersona}'", conexion);
registroPersona.ExecuteNonQuery();
actualizacionExitosa = true;
} catch(Exception error){
Console.WriteLine("Ocurrió un error (Método ActualizarTelefono): " + error.Message);
}finally{
conexion.Close();
}
return actualizacionExitosa;
}
//METODOS PRIVADOS PARA REALIZAR LAS INSERCIONES DE DATOS
private bool RegistrarNombre(string nombre){
bool registroExitoso = false;
try
{
conexion.Open();
var registroPersona = new MySqlCommand($"INSERT INTO Persona(Nombre) VALUES ('{nombre}');", conexion);
registroPersona.ExecuteNonQuery();
registroExitoso = true;
} catch(Exception error){
Console.WriteLine("Ocurrió un error (Método RegistrarNombre): " + error.Message);
}finally{
conexion.Close();
}
return registroExitoso;
}
private bool EliminarNombre(string idPersona){
bool eliminacionExitosa = false;
try
{
conexion.Open();
var comandoEliminar = new MySqlCommand($"DELETE FROM Persona WHERE idPersona='{idPersona}';", conexion);
comandoEliminar.ExecuteNonQuery();
eliminacionExitosa = true;
} catch(Exception error){
Console.WriteLine("Ocurrió un error (Método EliminarNombre): " + error.Message);
}finally{
conexion.Close();
}
return eliminacionExitosa;
}
private bool RegistrarCorreo(string correo, string idPersona){
bool registroExitoso = false;
try
{
conexion.Open();
var registroCorreo = new MySqlCommand($"INSERT INTO Correo(Correo,Persona_idPersona) VALUES ('{correo}','{idPersona}');", conexion);
registroCorreo.ExecuteNonQuery();
registroExitoso = true;
} catch(Exception error){
Console.WriteLine("Ocurrió un error (Método RegistrarCorreo): " + error.Message);
}finally{
conexion.Close();
}
return registroExitoso;
}
private bool EliminarCorreo(string idPersona){
bool eliminacionExitosa = false;
try
{
conexion.Open();
var comandoEliminar = new MySqlCommand($"DELETE FROM Correo WHERE Persona_idPersona='{idPersona}';", conexion);
comandoEliminar.ExecuteNonQuery();
eliminacionExitosa = true;
} catch(Exception error){
Console.WriteLine("Ocurrió un error (Método EliminarCorreo): " + error.Message);
}finally{
conexion.Close();
}
return eliminacionExitosa;
}
private bool RegistrarTelefono(string telefono, string idPersona){
bool registroExitoso = false;
try
{
conexion.Open();
var registroTelefono = new MySqlCommand($"INSERT INTO Telefono(Numero,Persona_idPersona) VALUES ('{telefono}','{idPersona}');", conexion);
registroTelefono.ExecuteNonQuery();
registroExitoso = true;
} catch(Exception error){
Console.WriteLine("Ocurrió un error (Método RegistrarTelefono): " + error.Message);
}finally{
conexion.Close();
}
return registroExitoso;
}
private bool EliminarTelefono(string idPersona){
bool eliminacionExitosa = false;
try
{
conexion.Open();
var comandoEliminar = new MySqlCommand($"DELETE FROM Telefono WHERE Persona_idPersona='{idPersona}';", conexion);
comandoEliminar.ExecuteNonQuery();
eliminacionExitosa = true;
} catch(Exception error){
Console.WriteLine("Ocurrió un error (Método EliminarCorreo): " + error.Message);
}finally{
conexion.Close();
}
return eliminacionExitosa;
}
private string ObtenerId(string nombreContacto){
string idObtenido = ID_PERSONA_ERROR;
try
{
conexion.Open();
var command = new MySqlCommand($"SELECT idPersona FROM Persona WHERE Nombre = '{nombreContacto}'", conexion);
var reader = command.ExecuteReader();
while (reader.Read())
{
idObtenido = reader.GetInt32(0).ToString();
}
}catch(Exception error){
Console.WriteLine("Ocurrió un error (Método ObtenerId)" + error.Message);
}finally{
conexion.Close();
}
return idObtenido;
}
}
} | 1e37aff5707fecec7c95a52b973ef97dc5bc6d7b | [
"C#",
"YAML",
"Dockerfile",
"SQL"
] | 6 | C# | BaezCrdrmUV/contenedores-1-AngelJuarezGarcia | cf1295069ccf6d40b1832345e3c0138633b011f8 | a726d930d73172a4feb825d526a86e724bbda86d | |
refs/heads/main | <file_sep>/*Just Check for jQuery work*/
//alert(33);
/* slideUp & slideDown Start*/
$(document).ready(function () {
$("#slideup").click(function () {
$("#fourth").slideUp(1000);
});
$("#slidedown").click(function () {
$("#fourth").slideDown(1000);
});
});
/* slideUp & slideDown End*/
/* slideToggle Start*/
$(document).ready(function () {
$("#slidetoggle").click(function () {
$("#fifth").slideToggle(1000);
});
});
/* slideToggle End*/
<file_sep>/*Show & Hide Start
1. Use click() Event function.
2. Use dblclick() Event function.
3. Use mouseover() Event function.
4. Use mouseout() Event function.
*/
jQuery(document).ready(function () {
jQuery("#hide").click(function () {
jQuery("#first").hide(1000);
});
jQuery("#show").click(function () {
jQuery("#first").show(1000);
});
});
/*Show & Hide End*/
/*Toggle Start*/
$(document).ready(function () {
$("#toggle").click(function () {
$("#second").toggle(1000);
});
});
/*Toggle End*/
/*Fadein and FadeOut Start*/
jQuery(document).ready(function () {
jQuery("#fadein").click(function () {
jQuery("#third").fadeIn(1000);
});
jQuery("#fadeout").click(function () {
jQuery("#third").fadeOut(1000);
});
});
/*Fadein and FadeOut End*/
<file_sep>$(document).ready(function(){
$("#hide").click(function(){
$(".first").hide(2000);
});
$("#show").click(function(){
$(".first").show(1000);
});
});<file_sep><!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>CSS Learning</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Deserunt, nobis.</h1>
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Omnis nostrum praesentium commodi dolor aut excepturi reprehenderit suscipit iusto consectetur, magni adipisci alias deleniti ex error expedita doloremque quia neque, distinctio, eos non saepe illum consequuntur. Reprehenderit quod, veniam nam. Hic ipsam, sapiente tempore dolores. Assumenda voluptatem nobis perspiciatis at vero nemo, fugiat veritatis ab sed, nulla cupiditate placeat aperiam ducimus facilis, repellendus voluptate. Consectetur asperiores aperiam dolore itaque a doloremque libero, non eaque. Sed unde enim necessitatibus adipisci doloribus! Accusamus debitis velit harum dolorem rerum molestias perferendis amet maxime sunt facere optio ipsam possimus, doloremque natus. Odit libero impedit laudantium velit aperiam cupiditate voluptatem officia esse quam illum eligendi culpa eaque tenetur quos, officiis architecto nam accusamus repellat repellendus vel, eos exercitationem magni laboriosam aliquam. Fugiat ab esse praesentium? Minima id deserunt dolorem saepe tempora, libero cupiditate repellat. Inventore dolorem numquam sapiente culpa nobis consequatur, quos sint asperiores id harum tenetur, aut. Officia ipsam perferendis, cumque culpa, beatae accusantium non explicabo at deserunt, vitae quia tenetur sit numquam dignissimos nihil vel impedit incidunt quod cupiditate. In temporibus vitae, hic! Consectetur deleniti nesciunt repellendus sequi porro deserunt veniam dolore beatae blanditiis mollitia! Ad perferendis voluptatum, enim nulla ipsa repellat est cupiditate veritatis voluptates distinctio molestias labore nemo quia iure. A deleniti doloremque quo eius, voluptatum neque sit re
</p>
</body>
</html><file_sep>$(document).ready(function () {
$(".para").mouseover(function () {
$(this).stop().animate({
width: "400px"
});
});
$(".para").mouseout(function(){
$(this).stop().animate({width: "300px"});
});
});
<file_sep><!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>MIET AMIE Engineering & ICT Park</title>
<meta name="author" content="<NAME>">
<meta name="description" content="Free Website tutorials and IT Training Center in Bangladesh">
<meta name="keywords" content="HTML, CSS,Bootstrap,Jquery,PHP,MySQl,Bangla website tutorials, XML, JavaScript,MIET Training Institute,web design, web development">
<meta http-equiv="refresh" content="300">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="stylesheet" href="css/bootstrap.min.css">
<link rel="stylesheet" href="css/font-awesome.min.css">
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<section id="main">
<!--
<section id="search">
<div class="container">
<form class="form-inline my-2 my-lg-0">
<input class="form-control mr-sm-2" type="search" placeholder="Search" aria-label="Search">
<button class="btn btn-outline-success my-2 my-sm-0" type="submit">Search</button>
</form>
</div>
</section>
-->
<section id="slider">
<div class="container">
<div id="carouselExampleIndicators" class="carousel slide" data-ride="carousel">
<ol class="carousel-indicators">
<li data-target="#carouselExampleIndicators" data-slide-to="0" class="active"></li>
<li data-target="#carouselExampleIndicators" data-slide-to="1"></li>
<li data-target="#carouselExampleIndicators" data-slide-to="2"></li>
</ol>
<div class="carousel-inner">
<div class="carousel-item active">
<img class="d-block w-100" src="imgs/banner.jpg" alt="First slide">
</div>
<div class="carousel-item">
<img class="d-block w-100" src="imgs/computer.jpg" alt="Second slide">
</div>
<div class="carousel-item">
<img class="d-block w-100" src="imgs/tech.jpg" alt="Third slide">
</div>
</div>
<a class="carousel-control-prev" href="#carouselExampleIndicators" role="button" data-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="sr-only">Previous</span>
</a>
<a class="carousel-control-next" href="#carouselExampleIndicators" role="button" data-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="sr-only">Next</span>
</a>
</div>
</div>
</section>
<section id="nav">
<div class="container">
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="#">MIET</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSupportedContent">
<ul class="navbar-nav mr-auto">
<li class="nav-item active">
<a class="nav-link" href="#">Home <span class="sr-only">(current)</span></a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Academic</a>
</li>
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" href="#" id="navbarDropdown" role="button" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false">AMIE Engineering</a>
<div class="dropdown-menu" aria-labelledby="navbarDropdown">
<a class="dropdown-item" href="#">Action</a>
<a class="dropdown-item" href="#">Another action</a>
<div class="dropdown-divider"></div>
<a class="dropdown-item" href="#">Something else here</a>
</div>
</li>
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" href="#" id="navbarDropdown" role="button" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false">Our Trainings</a>
<div class="dropdown-menu" aria-labelledby="navbarDropdown">
<a class="dropdown-item" href="#">Action</a>
<a class="dropdown-item" href="#">Another action</a>
<div class="dropdown-divider"></div>
<a class="dropdown-item" href="#">Something else here</a>
</div>
</li>
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" href="#" id="navbarDropdown" role="button" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false">Hostel</a>
<div class="dropdown-menu" aria-labelledby="navbarDropdown">
<a class="dropdown-item" href="#">Action</a>
<a class="dropdown-item" href="#">Another action</a>
<div class="dropdown-divider"></div>
<a class="dropdown-item" href="#">Something else here</a>
</div>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Admission</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">ICT</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Contact Us</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">About Us</a>
</li>
</ul>
</div>
</nav>
</div>
<section id="scroll">
<div class="container">
<marquee behavior="alternate" style="background-color:whitesmoke; color: green; padding: 10px; margin-bottom: 6px; font-size: 20px;">ভর্তি চলছে ! ভর্তি চলছে !! ভর্তি চলছে !!! আপনি কি ফ্রিল্যান্সিং এ ক্যারিয়ার গড়তে চান? আউটসোর্সিং ট্রেনিং নিয়ে আপনিও সাবলম্বী হউন। আমাদের কোর্সগুলোতে ভর্তি চলছে। দ্রুত যোগাযোগ করুন। অধ্যক্ষ : 01714 66 03 47</marquee>
</div>
</section>
<section>
<div class="container">
<div class="col-md-12">
<div class="content">
<h1 style="text-align: center;">Welcome to <span style="color: red; font-family: cursive">MIET</span><sup style="font-size: 16px; margin-left: 6px;font-weight: bold">®</sup></h1>
<p style="height: 300px; line-height: 22px;">
<b>Application Courses</b> - Beginner to advanced skills in major Computer sector<br>
<b>Technical Skills Courses</b> - Designed to advance your IT skills<br>
<b>Certification Courses</b> - Classes to help you prepare for BTEB certification<br><br>
<code style="font-size: 20px;">Imparted ICT training for the participants of different courses.</code><br><br>
<b><span style="color: red; font-family: cursive">MIET</span> AMIE Engineering</b><br><br>
<b><span style="color: red; font-family: cursive">MIET</span> Training Institute & ICT College</b><br><br>
<b><span style="color: red; font-family: cursive">MIET</span> Hostel</b>
</p>
</div>
</div>
<section>
<div class="container">
<hr style="width: 1000px; color: lawngreen">
</div>
</section>
<!--
<div class="col-md-6">
<div class="link">
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">Academic</a></li>
<li><a href="#">AMIE Engineering</a></li>
<li><a href="#">Admission</a></li>
<li><a href="#">ICT</a></li>
<li><a href="#">Our Trainings</a></li>
<li><a href="#">Gallery</a></li>
<li><a href="#">Hostel</a></li>
<li><a href="#">Cantact Us</a></li>
<li><a href="#">About</a></li>
</ul>
</div>
</div>
-->
</div>
</section>
<br>
<section id="course">
<div class="container">
<article>
<h1> ওয়েব ডিজাইন এবং ডেভেলপমেন্ট </h1>
<p>
ওয়েব ডিজাইন কি? ওয়েব ডিজাইন মানে হচ্ছে একটা ওয়েবসাইট দেখতে কেমন হবে বা এর সাধারন রূপ কেমন হবে তা নির্ধারণ করা। ওয়েব ডিজাইনার হিসেবে আপনার কাজ হবে একটা পূর্ণাঙ্গ ওয়েব সাইটের টেম্পলেট বানানো। যেমন ধরুন এটার লেয়াউট কেমন হবে। হেডারে কোথায় মেনু থাকবে, সাইডবার হবে কিনা, ইমেজগুলো কিভাবে প্রদর্শন করবে ইত্যাদি। ভিন্ন ভাবে বলতে গেলে ওয়েবসাইটের তথ্য কি হবে এবং কোথায় জমা থাকবে এগুলো চিন্তা না করে, তথ্যগুলো কিভাবে দেখানো হবে সেটা নির্ধারণ করাই হচ্ছে ওয়েব ডিজাইনার এর কাজ। আর এই ডিজাইন নির্ধারণ করতে ব্যাবহার করতে হবে কিছু প্রোগ্রামিং, স্ক্রিপ্টিং ল্যাঙ্গুয়েজ এবং মার্কআপ ল্যাঙ্গুয়েজ।
</p>
<p>ছয় মাসব্যাপী এ প্রশিক্ষণটিতে থাকছ</p>
<p style="margin-left: 100px;">এইচটিএমএল, সিএসএস<br> এসইচটিএমএল ৫, সিএসএস ৩<br> জাভাস্ক্রিপ্ট, জেকিউয়ারি<br> জেকোয়ারী প্লাগিন্স এর ব্যাবহার<br> পিএসডি টু এসইচটিএমএল ৫- সিএসএস ৩<br> ইউ আই কিট এর ব্যাবহার<br> বুটস্ট্র্যাপ এর ব্যাবহার<br> প্রফেশনাল পিএইচপি<br> ওয়ার্ডপ্রেস থিম ডেভেলপমেন্ট<br> ওয়ার্ডপ্রেস থিম কাস্টমাইজেশন<br> ওয়ার্ডপ্রেস প্লাগিন ডেভেলপমেন্ট<br> ওয়েব টেমপ্লেট তৈরি<br> রেসপনসিভ লেয়াউট ডিজাইন<br> এইচটিএমএল টু ওয়ার্ডপ্রেস কনভার্শন</p><br><br>
<p>আপওয়ার্ক , ফাইবার , পিপল পার আওয়ার এ প্রোফাইল ডেভেলপ করা, কাজে এপ্লাই করার টিপস এবং সিক্রেট,বিডিং টেকনিক। এছাড়াও প্রত্যেকটি বিষয়ে প্রফেশনাল সেক্টরে দক্ষ হাতে কলমে শেখানো সহ পূর্ণ সার্টিফাইড প্রফেশনাল ওয়ার্ডপ্রেস ডেভেলপমেন্ট শেখানো হবে। ফ্রিল্যান্সিং এ ক্যারিয়ার গড়তে রিয়েল প্রফেশনাল ওয়ার্ডপ্রেস ডেভেলপারদের কাছ থেকে শিখুন এবং নিজেকে আন্তর্জাতিক মানের দক্ষ হিসেবে নিশ্চিত করুন।</p>
<br>
<section>
<div class="container">
<hr style="width: 1000px; color: lawngreen">
</div>
</section>
<br>
<h1> গ্রাফিক্স ডিজাইন এবং মাল্টিমিডিয়া প্রোগ্রামিং </h1>
<p>ফ্রিল্যান্সিংয়ের মাধ্যমে অর্থ উপার্জনের অন্যতম একটি মাধ্যম হল গ্রাফিক্স ডিজাইন। গ্রাফিক্স ডিজাইন এমনি একটি ক্ষেত্র, যার চাহিদা দিন দিন বেড়েই চলছে। একজন প্রফেশনাল ডিজাইনার মাসে হাজার ডলারেরও উপরে আয় করতে পারেন। Odesk, Elance , Fiverr, Peopleperhou গ্রাফিক ডিজাইন প্রচুর কাজ পাওয়া যায় । যারা নতুন ফ্রিল্যান্সিং এর কাজ শিখতে চান তারা গ্রাফিক্স ডিজাইন এর কাজ শিখতে পারেন । বাংলাদেশেও এর চাহিদা অনেক রয়েছে। তবে নারীদের জন্য এটি একটি ভাল সেক্টর। আমার জানা মতে বাংলাদেশের অনেক নারী অনলাইনে গ্রাফিক্স ডিজাইনের কাজ করে প্রচুর টাকা আয় করছেন। তবে এ কাজ ভালো করে শিখতে হবে।<br> গ্রাফিক্স ডিজাইনের যেসব কাজ পাবেন ফ্রিল্যান্সিং মার্কেটপ্লেসগুলিতে…<br><br></p>
<p style="margin-left: 100px;">১. লোগো ডিজাইন<br> ২. বিজনেস কার্ড ডিজাইন<br> ৩. ব্যানার/পোস্টার ডিজাইন<br> ৪. ওয়েব সাইটের জন্য পিএসডি তৈরি<br> ৫. স্টিকার ডিজাইন<br> ৬. প্রোডাক্ট হলোগ্রাম ডিজাইন<br> ৭. ইমেজ এডিটিংএন্ড রিসাইজ<br> ৮. ফটো রিটাচিং<br> ৯. স্কেচ তৈরি/ড্রয়িং করা </p><br>
<p>
গ্রাফিক্স ডিজাইন এর একটি অংশ হচ্ছে লোগো ডিজাইন । তবে এটি ছাড়াও আরও অনেক কাজ রয়েছে গ্রাফিক্স ডিজাইনে। যেমন বিজনেস কার্ড ডিজাইন, ব্যানার/পোস্টার ডিজাইন,ওয়েব সাইটের জন্য পিএসডি তৈরি, স্টিকার ডিজাই, প্রোডাক্ট হলোগ্রাম ডিজাইন, ইমেজ এডিটিং এন্ড রিসাইজ ইত্যাদি। তবে ফ্রিল্যান্সিং মার্কেটপ্লেসগুলিতে ইন্টোরিয়র ডিজাইন এরও ব্যাপক চাহিদা রয়েছে ব্যানার, বিলবোর্ড থেকে শুরু করে বিভিন্ন কভার পেইজের ডিজাইন এর কাজও পাওয়া যায় অনলাইনে। । একটি ঘরের ভিতর বিভিন্ন অংশ কেমন হবে সেটা ডিজাইন করা। তাছাড়া বিভিন্ন দোকানের ডিজাইন এর কাজ পাওয়া যায়।
</p>
</article>
</div>
</section>
<section id="scroll-top">
<div class="container">
<p><a href="#" class="top">উপরে যান</a></p>
</div>
</section>
<section>
<div class="container">
<div class="footer">
<p>©Copyrighth 2014 - 2018 <span style="color: #B0FB31; font-family: cursive; font-size: 25px;">MIET</span>. All Right Reserved.<br><a href="http://www.mietbd.com" target="_blank"><i class="fa fa-desktop"></i></a><br>Developed By <code style="font-size: 20px;"><a href="https://www.fb.com/science.foysal" target="_blank" style="text-decoration: none; color: #B0FB31"><strong> <NAME></strong></a></code></p>
<div class="social">
<a href="https://www.facebook.com/miet.ict.help" target="_blank"><i class="fa fa-facebook"></i></a>
<a href="https://www.facebook.com/groups/miet.training/" target="_blank"><i class="fa fa-twitter"></i></a>
<a href="https://www.youtube.com/channel/UC99fC5VeD0p4sf5zAXigzJQ" target="_blank"><i class="fa fa-youtube"></i></a>
<a href="http://www.mietbd.com" target="_blank"><i class="fa fa-rss"></i></a>
<a href="01714 66 03 47" target="_blank"><i class="fa fa-phone"></i></a>
<a href="https://www.facebook.com/groups/miet.training/" target="_blank"><i class="fa fa-facebook"></i></a>
<i class="fa fa-wifi"></i>
</div>
</div>
</div>
</section>
</section>
<!--
<script src="https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity="<KEY>" crossorigin="anonymous"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js" integrity="<KEY>" crossorigin="anonymous"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js" integrity="<KEY>" crossorigin="anonymous"></script>
-->
<script src="js/jquery-3.3.1.min.js" type="text/javascript"></script>
<script src="js/bootstrap.min.js" type="text/javascript"></script>
<script src="js/script.js" type="text/javascript"></script>
</section>
</body>
</html>
<file_sep>/* slideToggle & stop() Start*/
$(document).ready(function () {
$("#slide").click(function () {
$("#one").slideToggle(3000);
});
$("#stop").click(function () {
$("#one").stop();
});
});
/* slideToggle & stop() End*/
<file_sep><!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Bangabandhu-1 Satellite</title>
<link rel="icon" href="imgs/me.jpg" sizes="16x16">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="stylesheet" href="css/font-awesome.min.css">
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<!--Header Section-->
<div class="header">
<div class="content">
<h2>Bangabandhu-1 Satellite</h2>
</div>
<div class="details">
<div class="one">
<img src="imgs/1.png" alt="" class="s">
<h1>বঙ্গবন্ধু-১</h1>
<p>বাংলাদেশের প্রথম স্যাটেলাইট (কৃত্রিম উপগ্রহ)</p>
<h3>উৎক্ষেপণ সময়: ১১ মে, ২০১৮</h3>
</div>
<div class="two">
<img src="imgs/govt-logo.png" alt="">
<img src="imgs/bd_flag.png" alt="" style="width: 150px; height: 110px;">
</div>
<div class="three">
<img src="imgs/2.png" alt="" class="w">
<h4>উত্পাদক: Thales Alenia Space</h4>
<h5>চুক্তিকারী: স্পেসএক্স</h5>
<p>বহনকারী রকেট: Falcon 9 Block 5</p>
<p>অবস্থান: ১১৯.১ ডিগ্রি পূর্ব দ্রাঘিমাংশে</p>
<p>মিশন সময়কাল: ১৫ বছর</p>
<p>প্রজেক্ট খরচ: তিন হাজার কোটি টাকা</p>
</div>
</div>
</div>
<div class="article">
<div class="first">
<h3>মহাকাশে যাত্রার চূড়ান্ত ক্ষণে বাংলাদেশের প্রথম স্যাটেলাইট বঙ্গবন্ধু-১।</h3>
<p>আজ বাংলাদেশ সময় দিবাগত রাত ২টা ১২ মিনিট থেকে ৪টা ২২ মিনিটের মধ্যে যেকোনো সময় স্যাটেলাইটটি উৎক্ষেপণ করা হতে পারে।<br><br>যুক্তরাষ্ট্রের ফ্লোরিডার কেনেডি স্পেস সেন্টার থেকে স্যাটেলাইটটি উৎক্ষেপণ করা হবে। উৎক্ষেপণ সফল হলে বিশ্বের ৫৭ তম দেশ হিসেবে নিজস্ব স্যাটেলাইটের মালিক হবে বাংলাদেশ। দেশের প্রত্যন্ত অঞ্চলে এই স্যাটেলাইটের মাধ্যমে ইন্টারনেট ও টেলিযোগাযোগ সেবার সম্প্রসারণ করা সম্ভব হবে। দুর্যোগ পরিস্থিতি মোকাবিলা ও ব্যবস্থাপনায় নতুন মাত্রা যোগ হবে। স্যাটেলাইটভিত্তিক টেলিভিশন সেবা ডিটিএইচ (ডিরেক্ট টু হোম) ও জাতীয় নিরাপত্তা নিশ্চিত করার কাজেও এ স্যাটেলাইটকে কাজে লাগানো যাবে। বঙ্গবন্ধু-১ স্যাটেলাইট উৎক্ষেপণের মুহূর্তটি বাংলাদেশ টেলিভিশনসহ দেশের সব কটি বেসরকারি টেলিভিশন সরাসরি সম্প্রচার করবে। দুর্লভ এ মুহূর্ত সরাসরি প্রচারের ব্যবস্থা নিতে মন্ত্রিপরিষদ বিভাগ দেশের সব জেলা ও উপজেলা প্রশাসনকে নির্দেশ দিয়েছে। বঙ্গবন্ধু স্যাটেলাইট উৎক্ষেপণকারী প্রতিষ্ঠান স্পেসএক্সও উৎক্ষেপণ মুহূর্তটি সরাসরি সম্প্রচার করবে। কেনেডি স্পেস সেন্টারের দুটি স্থান থেকে আগ্রহী দর্শনার্থীরা এই উৎক্ষেপণ দেখতে পারবেন। একটি স্থান অ্যাপোলো সেন্টার, উৎক্ষেপণস্থল থেকে দূরত্ব ৬ দশমিক ২৭ কিলোমিটার। উৎক্ষেপণের দৃশ্য কেনেডি স্পেস সেন্টারের মূল দর্শনার্থী ভবন (মেইন ভিসিটর কমপ্লেক্স) থেকেও দেখা যাবে। উৎক্ষেপণ স্থল থেকে এটির দূরত্ব ১২ কিলোমিটার।<br><br>স্যাটেলাইট উৎক্ষেপণের এ মুহূর্তের সাক্ষী হতে প্রধানমন্ত্রীর তথ্যপ্রযুক্তি উপদেষ্টা সজীব ওয়াজেদ জয়ের নেতৃত্ব একটি প্রতিনিধিদল কেনেডি স্পেস সেন্টারে উপস্থিত থাকবে। মহাকাশে বঙ্গবন্ধু-১ স্যাটেলাইটের অবস্থান হবে ১১৯ দশমিক ১ ডিগ্রি পূর্ব দ্রাঘিমাংশে। এই কক্ষপথ থেকে বাংলাদেশ ছাড়াও সার্কভুক্ত সব দেশ, ইন্দোনেশিয়া, ফিলিপাইন, মিয়ানমার, তাজিকিস্তান, কিরগিজস্তান, উজবেকিস্তান, তুর্কমেনিস্তান ও কাজাখস্তানের কিছু অংশ এই স্যাটেলাইটের আওতায় আসবে। দেশের প্রথম এ স্যাটেলাইট তৈরিতে খরচ ধরা হয় ২ হাজার ৯৬৭ কোটি টাকা। এর মধ্যে ১ হাজার ৩১৫ কোটি টাকা বাংলাদেশ সরকার ও বাকি ১ হাজার ৬৫২ কোটি টাকা ঋণ হিসেবে নেওয়া হয়েছে। এ ঋণ দিয়েছে বহুজাতিক ব্যাংক এইচএসবিসি। তবে শেষ পর্যন্ত প্রকল্পটি বাস্তবায়নে খরচ হয়েছে ২ হাজার ৭৬৫ কোটি টাকা। স্যাটেলাইট তৈরির এই পুরো কর্মযজ্ঞ বাস্তবায়িত হয়েছে বাংলাদেশ টেলিযোগাযোগ নিয়ন্ত্রণ কমিশনের (বিটিআরসি) তত্ত্বাবধানে। তিনটি ধাপে এই কাজ হয়েছে। এগুলো হলো স্যাটেলাইটের মূল কাঠামো তৈরি, স্যাটেলাইট উৎক্ষেপণ ও ভূমি থেকে নিয়ন্ত্রণের জন্য গ্রাউন্ড স্টেশন তৈরি। বঙ্গবন্ধু-১ স্যাটেলাইটের মূল অবকাঠামো তৈরি করেছে ফ্রান্সের মহাকাশ সংস্থা থ্যালেস অ্যালেনিয়া স্পেস।
<br><br> স্যাটেলাইট তৈরির কাজ শেষে গত ৩০ মার্চ এটি উৎক্ষেপণের জন্য যুক্তরাষ্ট্রের ফ্লোরিডায় পাঠানো হয়। সেখানে আরেক মহাকাশ গবেষণা সংস্থা স্পেসএক্সের ‘ফ্যালকন-৯’ রকেটে করে স্যাটেলাইটটি আজ মহাকাশে যেতে পারে। স্যাটেলাইট তৈরি এবং ওড়ানোর কাজটি বিদেশে হলেও এটি নিয়ন্ত্রণ করা হবে বাংলাদেশ থেকেই। এ জন্য গাজীপুরের জয়দেবপুরে তৈরি গ্রাউন্ড কনট্রোল স্টেশন (ভূমি থেকে নিয়ন্ত্রণব্যবস্থা) স্যাটেলাইট নিয়ন্ত্রণের মূল কেন্দ্র হিসেবে কাজ করবে। আর বিকল্প হিসেবে ব্যবহার করা হবে রাঙামাটির বেতবুনিয়া গ্রাউন্ড স্টেশন। শুরুর ইতিহাস বাংলাদেশে প্রথম স্যাটেলাইট নিয়ে কাজ শুরু হয় ২০০৭ সালে। সে সময় মহাকাশের ১০২ ডিগ্রি পূর্ব দ্রাঘিমাংশে কক্ষপথ বরাদ্দ চেয়ে জাতিসংঘের অধীন সংস্থা আন্তর্জাতিক টেলিযোগাযোগ ইউনিয়নে (আইটিইউ) আবেদন করে বাংলাদেশ। কিন্তু বাংলাদেশের ওই আবেদনের ওপর ২০টি দেশ আপত্তি জানায়। এই আপত্তির বিষয়টি এখনো সমাধান হয়নি। এরপর ২০১৩ সালে রাশিয়ার ইন্টারস্পুটনিকের কাছ থেকে বঙ্গবন্ধু-১ স্যাটেলাইটের বর্তমান কক্ষপথটি কেনা হয়। বাংলাদেশ বারবার আইটিইউর কাউন্সিল সদস্য নির্বাচিত হয়ে নীতিনির্ধারক পর্যায়ে থাকলেও এখন পর্যন্ত নিজস্ব কক্ষপথ আনতে পারেনি।<br> জাতিসংঘের মহাকাশবিষয়ক সংস্থা ইউনাইটেড নেশনস অফিস ফর আউটার স্পেস অ্যাফেয়ার্সের (ইউএনওওএসএ) হিসাবে, ২০১৭ সাল পর্যন্ত মহাকাশে স্যাটেলাইটের সংখ্যা ৪ হাজার ৬৩৫। প্রতিবছরই স্যাটেলাইটের এ সংখ্যা ৮ থেকে ১০ শতাংশ হারে বাড়ছে। এসব স্যাটেলাইটের কাজের ধরনও একেক রকমের। বঙ্গবন্ধু-১ স্যাটেলাইটটি বিভিন্ন ধরনের মহাকাশ যোগাযোগের কাজে ব্যবহার করা হবে। এ ধরনের স্যাটেলাইটকে বলা হয় ‘জিওস্টেশনারি কমিউনিকেশন স্যাটেলাইট’।
<br><br>পৃথিবীর ঘূর্ণনের সঙ্গে সঙ্গে এ স্যাটেলাইট মহাকাশে ঘুরতে থাকে। দেশের প্রথম ন্যানো স্যাটেলাইট ব্র্যাক অন্বেষা প্রকল্পের প্রিন্সিপাল ইনভেস্টিগেটর খলিলুর রহমান বলেন, ‘আমাদের ছেলে-মেয়েরা এখন নিজেরাই স্যাটেলাইট বানানোর দক্ষতা অর্জন করেছে। সরকারের সহযোগিতা পেলে ২০২১ সালের মধ্যে দেশেই নিজস্ব যোগাযোগ স্যাটেলাইট তৈরি করা সম্ভব। বঙ্গবন্ধু স্যাটেলাইট তৈরি না হলে এসবের কিছুই হতো না।’ বর্তমানে দেশে প্রায় ৩০টি স্যাটেলাইট টেলিভিশন চ্যানেল সম্প্রচারে আছে। এসব চ্যানেল সিঙ্গাপুরসহ বিশ্বের বিভিন্ন দেশ থেকে স্যাটেলাইট ভাড়া নিয়ে পরিচালিত হচ্ছে। সব মিলিয়ে স্যাটেলাইটের ভাড়া বাবদ বছরে চ্যানেলগুলোর খরচ হয় ২০ লাখ ডলার বা প্রায় ১৭ কোটি টাকা। বঙ্গবন্ধু স্যাটেলাইট চালু হলে এই স্যাটেলাইট ভাড়ার অর্থ দেশেই থেকে যাবে। আবার স্যাটেলাইটের ট্রান্সপন্ডার বা সক্ষমতা অন্য দেশের কাছে ভাড়া দিয়েও বৈদেশিক মুদ্রা আয় করার সুযোগ থাকবে। এই স্যাটেলাইটের ৪০টি ট্রান্সপন্ডারের মধ্যে ২০টি ভাড়া দেওয়ার জন্য রাখা হবে। মহাকাশে যে কক্ষপথে বঙ্গবন্ধু স্যাটেলাইট স্থাপন করা হবে, তা দেশের টেলিভিশন চ্যানেলগুলোর জন্য ব্যবহার করা কঠিন হবে বলে মনে করেন বেসরকারি টেলিভিশন চ্যানেল একাত্তর টিভির ব্যবস্থাপনা পরিচালক ও প্রধান সম্পাদক মোজাম্মেল বাবু। তিনি প্রথম আলোকে বলেন, বঙ্গবন্ধু স্যাটেলাইট মহাকাশের যেখানে স্থাপন করা হবে, তা দিয়ে কাজ করা বাংলাদেশের টেলিভিশনগুলোর জন্য হবে একটি চ্যালেঞ্জ।<br><br> টেলিভিশন চ্যানেলগুলো যাতে এ স্যাটেলাইটের সুবিধা পায়, সে জন্য যা দরকার তা করতে হবে। এ বিষয়ে বিটিআরসির চেয়ারম্যান শাহজাহান মাহমুদ গত সোমবার যুক্তরাষ্ট্রে যাওয়ার আগে প্রথম আলোকে বলেন, বঙ্গবন্ধু স্যাটেলাইট ব্যবহারে দেশীয় চ্যানেলগুলোর কোনো সমস্যা হলে তা সমাধান করা হবে। বিটিআরসি নতুন প্রযুক্তির প্রতি খুবই উদার। এ বিষয়ে ব্যবহারকারীদের যেকোনো প্রস্তাব বিবেচনা করা হবে। তিনি আরও বলেন, আবহাওয়া ঠিক থাকলে স্যাটেলাইটটি আজ রাতের নির্ধারিত সময়েই উৎক্ষেপণ হবে।<br>___প্রথম আলো</p>
<hr>
<br><br>
</div>
</div>
</div>
<div class="me">
<h2><NAME></h2>
<p>Web Developer</p>
<address>
Satkhira,Khulna,Bangladesh
</address>
<br>
<br>
<div class="social">
<div class="f"><a href="https://fb.com/gelabi.alam" target="_blank"><i class="fa fa-facebook" aria-hidden="true"></i></a></div>
<div class="y">
<a href="https://www.youtube.com/ComputerEngineeringTutorials" target="_blank"><i class="fa fa-youtube" aria-hidden="true"></i></a>
</div>
<div class="z">
<a href="https://www.youtube.com/ComputerEngineeringTutorials" target="_blank"><i class="fa fa-youtube" aria-hidden="true"></i></a>
</div>
</div>
</div>
</body>
</html>
| bbabc0091828603feb3d34e24c0a0898a5d03f26 | [
"JavaScript",
"HTML"
] | 8 | JavaScript | gelabi-alam/Website-Development-Practise | d2b02536c8ae19977d0eed2a8864e87e3dd7d099 | 7431a46ece7db7a4275281449d1f57666f8bbe66 | |
refs/heads/master | <file_sep>var blocks = new Array(20);
var model = new Array(20);
// piece[0]-piece[3] are block positions, piece[4] is piece type
var piece = new Array(5);
var oldPos = new Array(4);
var display;
var speed = 1500;
var type;
var colors = ['-', 'i', 'j', 'l', 'o', 's', 'z', 't'];
var paused = false;
window.onload = function() {
setUp();
printGrid();
start();
}
function start() {
piece[0] = [-1, 5];
newPiece();
display = setInterval(play, speed);
}
function play() {
document.getElementById("pause").onclick = pause;
document.getElementById("reset").onclick = reset;
document.onkeydown = function(event) {
if (!paused){
switch (event.keyCode) {
case 39:
move(0, 1);
break;
case 38:
rotatePiece()
break;
case 37:
move(0, -1);
break;
case 40:
move(1, 0);
break;
case 32:
clearInterval(display);
while (move(1, 0) == true) continue;
checkRows();
if (!gameOver()) {
start();
} else {
updatePos();
printGrid();
resetModel();
start();
}
}
}
};
if (!paused) {
if (move(1, 0) == false) {
clearInterval(display);
checkRows();
if (!gameOver()) {
start();
} else {
updatePos();
printGrid();
resetModel();
start();
}
}
}
}
function pause() {
paused = !paused;
if (paused) {
document.getElementById("pause").innerHTML = 'RESUME';
document.getElementById("pause").style.color = '#a6898a';
document.getElementById("pause").style.borderColor = '#a6898a';
} else {
document.getElementById("pause").innerHTML = 'PAUSE'
document.getElementById("pause").style.color = '#c6b5b7';
document.getElementById("pause").style.borderColor = '#dbd7d7';
}
document.getElementById("pause").blur();
return;
}
function reset() {
document.getElementById("reset").blur();
if (paused) {
paused = !paused;
document.getElementById("pause").innerHTML = 'PAUSE'
document.getElementById("pause").style.color = '#c6b5b7';
document.getElementById("pause").style.borderColor = '#dbd7d7';
}
clearInterval(display);
resetModel();
start();
}
// returns true if piece is successfully moved, otherwise returns false
function move(x, y) {
oldPos = piece.concat();
clearPiece();
for (var i = 0; i < 4; i++)
piece[i] = [piece[i][0] + 1, piece[i][1] + y];
if (checkOverlap() == false) {
updatePos();
printGrid();
return true;
} else {
piece = oldPos.concat();
updatePos();
return false;
}
}
// called from movePiece() and gameOver()
function checkOverlap() {
for (var i = 0; i < 4; i++) {
if (piece[i][0] < 0) continue;
// check active blocks against bottom
if (piece[i][0] >= 20) return true;
// check active blocks against locked blocks
if (model[piece[i][0]][piece[i][1]] != 0) return true;
// check active blocks against sides
if (piece[i][1] < 0 || piece[i][1] > 9) return true;
}
return false;
}
function clearPiece() {
for (var i = 0; i < 4; i++)
if (piece[i][0] >= 0 && piece[i][1] >= 0)
model[oldPos[i][0]][oldPos[i][1]] = 0;
}
function updatePos() {
for (var i = 0; i < 4; i++)
if (piece[i][0] >= 0 && piece[i][0] <= 20)
model[piece[i][0]][piece[i][1]] = piece[4];
}
// called from play()
function rotatePiece() {
oldPos = piece.concat();
clearPiece();
for (var i = 1; i < 4; i++) {
y = piece[i][0] - piece[0][0];
x = piece[i][1] - piece[0][1];
x *= -1;
piece[i] = [piece[0][0] + x, piece[0][1] + y];
}
if (checkOverlap() == false) {
updatePos();
printGrid();
} else {
piece = oldPos.concat();
updatePos();
}
}
function checkRows() {
var rows = Array();
var row_count = 0;
for (var i = 19; i >= 0; i--) {
for (var j = 0; j < 10; j++)
if (model[i][j] != 0) row_count++;
if (row_count == 0) break;
if (row_count >= 10) rows.push(i);
row_count = 0;
}
if (rows.length > 0) deleteRows(rows);
}
function deleteRows(rows) {
deleted = 0;
for (var i = rows[0]; i >= 0; i--) {
if (i >= deleted) {
if (rows.includes(i)) {
deleted++;
} else {
model[i+deleted] = model[i].concat();
}
} else {
model[i] = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0];
}
}
printGrid();
}
// called from main function
function gameOver() {
for (var i = 0; i < 4; i++)
if (piece[i][0] < 0) return true;
return false;
}
// called from main function
function printGrid() {
for (var i = 0; i < 20; i++) {
for (var j = 0; j < 10; j++) {
blocks[i][j].classList.remove('i', 'l', 'j', 'o', 's', 'z', 't');
if (model[i][j] == 0) {
blocks[i][j].style.display = "none";
} else {
blocks[i][j].style.display = "block";
blocks[i][j].classList.add(colors[model[i][j]]);
}
}
}
}
function newPiece() {
piece[4] = 1 + Math.floor(Math.random() * 7);
if (piece[4] == 1) {
// I-block
piece[1] = [piece[0][0] + 1, piece[0][1]];
piece[2] = [piece[0][0] - 1, piece[0][1]];
piece[3] = [piece[0][0] - 2, piece[0][1]];
} else if (piece[4] == 2) {
// J-block
piece[1] = [piece[0][0], piece[0][1] - 1];
piece[2] = [piece[0][0] - 1, piece[0][1]];
piece[3] = [piece[0][0] - 2, piece[0][1]];
} else if (piece[4] == 3) {
// L-block
piece[1] = [piece[0][0], piece[0][1] + 1];
piece[2] = [piece[0][0] - 1, piece[0][1]];
piece[3] = [piece[0][0] - 2, piece[0][1]];
} else if (piece[4] == 4) {
// O-block
piece[1] = [piece[0][0], piece[0][1] - 1];
piece[2] = [piece[0][0] - 1, piece[0][1]];
piece[3] = [piece[0][0] - 1, piece[0][1] - 1];
} else if (piece[4] == 5) {
// S-block
piece[1] = [piece[0][0] - 1, piece[0][1]];
piece[2] = [piece[0][0], piece[0][1] - 1];
piece[3] = [piece[0][0] - 1, piece[0][1] + 1];
} else if (piece[4] == 6) {
// Z-block
piece[1] = [piece[0][0], piece[0][1] + 1];
piece[2] = [piece[0][0] - 1, piece[0][1]];
piece[3] = [piece[0][0] - 1, piece[0][1] - 1];
} else {
// T-block
piece[1] = [piece[0][0], piece[0][1] - 1];
piece[2] = [piece[0][0], piece[0][1] + 1];
piece[3] = [piece[0][0] - 1, piece[0][1]];
}
}
function resetModel() {
for (var i = 0; i < 20; i++) {
model[i] = [ 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 ];
}
printGrid();
}
function setUp() {
title();
var grid = document.getElementById("grid");
for (var i = 0; i < 20; i++) {
// initializing model array
model[i] = new Array(10);
// initializing block array
blocks[i] = new Array(10);
for (var j = 0; j < 10; j++) {
// initializing model array
model[i][j] = 0;
// initializing block array
var col = j;
var row = i;
var block = document.createElement("div");
block.classList.add("block");
grid.appendChild(block);
block.style.left = col * 25 + "px";
block.style.top = row * 25 + "px";
blocks[i][j] = block;
}
}
}
function title() {
var area = document.getElementById("title");
var rows = new Array(5);
rows[0] = [0, 1, 2, 4, 5, 7, 8, 9, 11, 12, 15, 18, 19];
rows[1] = [1, 4, 8, 11, 13, 15, 17];
rows[2] = [1, 4, 5, 8, 11, 12, 15, 18];
rows[3] = [1, 4, 8, 11, 13, 15, 19];
rows[4] = [1, 4, 5, 8, 11, 13, 15, 17, 18];
for (var i = 0; i < 5; i++) {
for (var j = 0; j < 20; j++) {
if (rows[i].includes(j)) {
var block = document.createElement("div");
block.classList.add(colors[i+2]);
area.appendChild(block);
block.style.left = j * 14 + "px";
block.style.top = i * 14 + "px";
}
}
}
}<file_sep># javascript-tetris
Tetris game I made for a web development course, written in javascript
| d71b4ac25b527091b7b084b62a5335d21f9928bb | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | jquish/javascript-tetris | cbef8ccbb2b11e8ccc385230e4f311ac6ceb7494 | dd5823d612d2511b4c5c08fc4357e820430b3298 | |
refs/heads/main | <repo_name>StevenLikeWatermelon/vue-shop-template<file_sep>/src/router/index.js
import Vue from 'vue'
import VueRouter from 'vue-router'
import Frame from '@/layout/Frame.vue'
Vue.use(VueRouter)
export const routes = [
{
path: '/',
name: 'frame',
redirect: '/guide',
component: Frame,
children: [
{
path: '/guide',
name: 'guide',
hiddden: true,
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/guide/index.vue')
},
{
path: '/home',
name: 'home',
meta: {
title: '首页'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/home/Home.vue')
},
{
path: '/search',
name: 'search',
meta: {
title: '搜索'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/search/index.vue')
},
{
path: '/detail',
name: 'detail',
meta: {
noTop: true,
title: '商品详情'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/detail/index.vue')
},
{
path: '/myOrder',
name: 'myOrder',
meta: {
noTop: true,
title: '订单详情'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/myOrder/index.vue')
},
{
path: '/orderSuccess',
name: 'orderSuccess',
meta: {
noTop: true,
title: '订单提交成功'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/orderSuccess/index.vue')
},
{
path: '/pay',
name: 'pay',
meta: {
noTop: true,
title: '结算单'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/pay/index.vue')
},
{
path: '/myOwnOrder',
name: 'myOwnOrder',
meta: {
noTop: true,
title: '我的订单'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/myOwnOrder/index.vue')
},
{
path: '/invoiceInfo',
name: 'invoiceInfo',
meta: {
noTop: true,
title: '发票信息'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/invoiceInfo/index.vue')
},
{
path: '/invoiceOperate',
name: 'invoiceOperate',
meta: {
noTop: true,
title: '开票操作'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/invoiceOperate/index.vue')
},
{
path: '/invoiceApply',
name: 'invoiceApply',
meta: {
noTop: true,
title: '开票申请'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/invoiceApply/index.vue')
},
{
path: '/myCar',
name: 'myCar',
meta: {
noTop: true,
title: '我的购物车'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/myCar/index.vue')
},
{
path: '/sendAdress',
name: 'sendAdress',
meta: {
noTop: true,
title: '收货地址'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/sendAdress/index.vue')
},
{
path: '/componeyInfo',
name: 'componeyInfo',
meta: {
noTop: true,
title: '公司信息'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/componeyInfo/index.vue')
},
{
path: '/myCoupon',
name: 'myCoupon',
meta: {
noTop: true,
title: '我的优惠券'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/myCoupon/index.vue')
},
{
path: '/myZGB',
name: 'myZGB',
meta: {
noTop: true,
title: '我的中光币'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/myZGB/index.vue')
},
{
path: '/settings',
name: 'settings',
meta: {
noTop: true,
title: '系统设置'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/settings/index.vue')
},
{
path: '/modal',
name: 'modal',
meta: {
noTop: true,
title: '弹框'
},
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '@/views/modal/index.vue')
}
]
}
]
const router = new VueRouter({
routes
})
export default router
<file_sep>/README.md
# vue-shop-template
vue-shop-template
| f68b8f129e4cea631f593bbce6c845ebed077fae | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | StevenLikeWatermelon/vue-shop-template | 9d1d1acafd239fd4b6db1dddb58bf3a65492c855 | 52274df877648c612d6a5822b094edca6262a209 | |
refs/heads/master | <repo_name>pdarkness/Doodle-Jump-clone<file_sep>/app/scripts/coin.js
/**
* Created with JetBrains WebStorm.
* User: knuturm
* Date: 10.9.2013
* Time: 14:29
* To change this template use File | Settings | File Templates.
*/
define(function(rect) {
var Coin = function(rect) {
this.rect = rect;
this.rect.right = rect.x + rect.width;
this.el = $('<div class="coin">');
this.el.css({
left: rect.x,
top: rect.y,
width: rect.width,
height: rect.height
});
};
Coin.prototype.onFrame = function() {}
return Coin;
});
<file_sep>/app/scripts/game.js
/*global define, $ */
define(['player', 'platform', 'coin', 'enemy', 'controls'], function(Player, Platform, Coin, Enemy, controls) {
var VIEWPORT_PADDING = 220;
/**
* Main game class.
* @param {Element} el DOM element containig the game.
* @constructor
*/
var Game = function(el) {
this.el = el;
this.player = new Player(this.el.find('.player'), this);
this.entities = [];
this.platformsEl = el.find('.platforms');
this.entitiesEl = el.find('.entities');
this.coinsEl = el.find('.coins');
this.worldEl = el.find('.world');
this.gameOvEl = el.find('.gameOver');
this.isPlaying = false;
this.gameScore = 0;
this.level = 1;
this.bonus = 0;
this.worldChunkSize = 1000;
this.worldFromY = 0;
this.worldToY = 1000;
this.nextCreatePlatformsY = 800;
this.sound = new Howl({
urls: ['/sounds/jump.mp3', '/sounds/jump.ogg']
})
// Cache a bound onFrame since we need it each frame.
this.onFrame = this.onFrame.bind(this);
};
Game.prototype.freezeGame = function() {
this.isPlaying = false;
};
Game.prototype.unFreezeGame = function() {
if (!this.isPlaying) {
this.isPlaying = true;
// Restart the onFrame loop
this.lastFrame = +new Date() / 1000;
requestAnimFrame(this.onFrame);
}
};
Game.prototype.createWorld = function() {
// Ground
for(i=0;i<10-this.level;i++){
//console.log(this.level(this.level));
this.addPlatform(new Platform({
x: 0,
y: -i*100,
width: 400,
height: 10
}));
}
};
Game.prototype.addPlatform = function(platform) {
this.entities.push(platform);
this.platformsEl.append(platform.el);
};
Game.prototype.addCoin = function(coin) {
this.entities.push(coin);
this.coinsEl.append(coin.el);
};
Game.prototype.addEnemy = function(enemy) {
this.entities.push(enemy);
this.entitiesEl.append(enemy.el);
};
Game.prototype.gameOver = function() {
this.freezeGame();
if (this.gameScore === 0){
alert('Game Over! Score: ' + Math.floor(this.player.maxScore));
}
else{
alert('Game Over! Score: ' + (this.gameScore+ Math.floor(this.player.maxScore)));
}
this.worldChunkSize = 1000;
this.worldFromY = 0;
this.worldToY = 1000;
this.nextCreatePlatformsY = 800;
var game = this;
setTimeout(function() {
game.start();
}, 0);
};
/**
* Runs every frame. Calculates a delta and allows each game entity to update itself.
*/
Game.prototype.onFrame = function() {
if (!this.isPlaying) {
return;
}
//if(this.nextCreatePlatformsY < this.player.pos.y)
if(Math.abs(this.player.pos.y)>this.nextCreatePlatformsY){
this.nextCreatePlatformsY += this.worldChunkSize;
this.worldFromY += this.worldChunkSize;
this.worldToY += this.worldChunkSize;
for(i=0;i<20;i++){
this.addPlatform(new Platform({
x: Math.floor(Math.random() * (380+ (i+1)))%380,
y: -Math.floor(Math.random() * (this.worldToY - this.worldFromY + 1) + this.worldFromY),
width: 80,
height: 10
}));
}
var mod = (Math.random()*1000)%400;
var modY = (-Math.floor(Math.random() * (this.worldToY - this.worldFromY + 1) + this.worldFromY));
this.addEnemy(new Enemy({
start: {x: mod, y: modY},
end: {x: mod-150, y: modY}
}));
this.addCoin(new Coin({
x: Math.floor(Math.random() * (380+ (i+1)))%380,
y: modY
}));
}
var now = +new Date() / 1000,
delta = now - this.lastFrame;
this.lastFrame = now;
controls.onFrame(delta);
this.player.onFrame(delta);
for (var i = 0, e; e = this.entities[i]; i++) {
e.onFrame(delta);
if (e.dead) {
this.entities.splice(i--, 1);
}
}
this.updateViewport();
// Request next frame.
requestAnimFrame(this.onFrame);
};
Game.prototype.updateViewport = function() {
var minY = this.viewport.y + VIEWPORT_PADDING;
var maxY = this.viewport.y + this.viewport.height + VIEWPORT_PADDING;
var playerY = this.player.pos.y;
var playerX = this.player.pos.x;
if (playerY < minY) {
this.viewport.y = playerY - VIEWPORT_PADDING;
} else if (playerY > maxY) {
this.viewport.y = playerY - this.viewport.height + VIEWPORT_PADDING;
}
this.worldEl.css({
left: -this.viewport.x,
top: -this.viewport.y
});
};
/**
* Starts the game.
*/
Game.prototype.start = function() {
// Cleanup last game.
this.entities.forEach(function(e) { e.el.remove(); });
this.entities = [];
// Set the stage.
this.createWorld();
this.player.reset();
this.viewport = {x: 0, y: 0, width: 400, height: 550};
// Then start.
this.unFreezeGame();
};
Game.prototype.forEachCoin = function(handler) {
for (var i = 0, e; e = this.entities[i]; i++) {
if (e instanceof Coin) {
handler(e);
}
}
};
Game.prototype.forEachPlatform = function(handler) {
for (var i = 0, e; e = this.entities[i]; i++) {
if (e instanceof Platform) {
handler(e);
}
}
};
Game.prototype.forEachEnemy = function(handler) {
for (var i = 0, e; e = this.entities[i]; i++) {
if (e instanceof Enemy) {
handler(e);
}
}
};
/**
* Cross browser RequestAnimationFrame
*/
var requestAnimFrame = (function() {
return window.requestAnimationFrame ||
window.webkitRequestAnimationFrame ||
window.mozRequestAnimationFrame ||
window.oRequestAnimationFrame ||
window.msRequestAnimationFrame ||
function(/* function */ callback) {
window.setTimeout(callback, 1000 / 60);
};
})();
return Game;
});<file_sep>/README.md
Doodle-Jump-clone
=================
My first html5 game =)<br>
clone repo<br>
npm install<br>
grunt server<br>
<br>
and play =)
<file_sep>/app/scripts/player.js
/*global define */
define(['controls'], function(controls) {
var PLAYER_SPEED = 350;
var JUMP_VELOCITY = 1450;
var GRAVITY = 4000;
var PLAYER_HALF_WIDTH = 14;
var PLAYER_RADIUS = 30;
var Player = function(el, game) {
this.game = game;
this.el = el;
controls.on('super', this.onSuper.bind(this));
};
Player.prototype.reset = function() {
this.pos = { x: 100, y: 0 };
this.vel = { x: 0, y: 0 };
this.playerScore = 0;
this.maxScore = 0;
}
Player.prototype.onFrame = function(delta) {
// Player input
this.vel.x = controls.inputVec.x * PLAYER_SPEED;
// Jumping
if ( this.vel.y === 0) {
this.vel.y = -JUMP_VELOCITY;
if(controls.keys.space)
this.vel.y -= 300;
if(controls.keys.down)
this.vel.y += 500;
this.game.sound.play();
}
//console.log(this.pos.y);
if (this.pos.x < 0)
this.pos.x = 400;
if (this.pos.x > 400)
this.pos.x = 0;
//console.log(this.pos.y);
//console.log(HELL_Y - this.maxScore);
// Gravity
this.vel.y += GRAVITY * delta;
var oldY = this.pos.y;
this.pos.x += delta * this.vel.x;
this.pos.y += delta * this.vel.y;
this.playerScore = Math.abs(oldY);
this.checkCoins(oldY);
if (this.playerScore > this.maxScore)
this.maxScore = this.playerScore;
// Collision detection
this.checkPlatforms(oldY);
this.checkEnemies();
this.checkGameOver();
// Update UI
this.el.css('transform', 'translate3d(' + this.pos.x + 'px,' + this.pos.y + 'px,0)');
this.el.toggleClass('left', this.vel.x < 0);
this.el.toggleClass('right', this.vel.x > 0);
this.el.toggleClass('jumping', this.vel.y < 0);
};
Player.prototype.onSuper = function () {
this.vel.y -= 300;
}
Player.prototype.checkGameOver = function() {
/*if (this.pos.y > HELL_Y - Math.floor(this.maxScore)) {
this.game.gameOver();
}*/
if (this.game.viewport.y != 0 && (this.pos.y- this.game.viewport.y)>this.game.viewport.height){
this.game.gameOver();
}
/*if (this.pos.y < 0) {
this.game.levelOver();
}*/
};
Player.prototype.checkPlatforms = function(oldY) {
var that = this;
this.game.forEachPlatform(function(p) {
// Are we crossing Y.
if (p.rect.y >= oldY && p.rect.y < that.pos.y) {
// Are inside X bounds.
if (that.pos.x + PLAYER_HALF_WIDTH >= p.rect.x && that.pos.x - PLAYER_HALF_WIDTH <= p.rect.right) {
// COLLISION. Let's stop gravity.
that.pos.y = p.rect.y;
that.vel.y = 0;
}
}
});
};
Player.prototype.checkCoins = function(oldY) {
var that = this;
this.game.forEachCoin(function(p) {
// Are we crossing Y.
if (p.rect.y >= oldY && p.rect.y < that.pos.y) {
// Are inside X bounds.
if (that.pos.x + PLAYER_HALF_WIDTH >= p.rect.x && that.pos.x - PLAYER_HALF_WIDTH <= p.rect.right) {
that.playerScore += 5000;
}
}
});
};
Player.prototype.checkEnemies = function() {
var centerX = this.pos.x;
var centerY = this.pos.y - 40;
var that = this;
this.game.forEachEnemy(function(enemy) {
// Distance squared
var distanceX = enemy.pos.x - centerX;
var distanceY = enemy.pos.y - centerY;
// Minimum distance squared
var distanceSq = distanceX * distanceX + distanceY * distanceY;
var minDistanceSq = (enemy.radius + PLAYER_RADIUS) * (enemy.radius + PLAYER_RADIUS);
// What up?
if (distanceSq < minDistanceSq) {
that.game.gameOver();
}
});
};
return Player;
});
| b89514f44aa7033023458f77611c2024befba17d | [
"JavaScript",
"Markdown"
] | 4 | JavaScript | pdarkness/Doodle-Jump-clone | ce11a9416f213cda3118cf89aae855bcf6bd4804 | c11cfe2df6aa19cf3cac3d98a91b7f7f4abfa199 | |
refs/heads/master | <file_sep>def square_array(array)
# your code here
arr = []
array.each do |square|
arr << square**2
end
return arr
end
| a24d1b7c8728ed0f47563ad1f4eb9b9c14c6a76d | [
"Ruby"
] | 1 | Ruby | raaynaldo/square_array-ruby-apply-000 | b495ab254c3264d849d122de8e1bd534c53ca416 | 341ca59a482c83614c87c967c9933ed1229198dc | |
refs/heads/master | <repo_name>jakubgs/netdev<file_sep>/kernel/fo.h
#ifndef _FO_H
#define _FO_H
#include <linux/fs.h> /* iovec, kiocb, file, pool_table_struct */
extern struct file_operations netdev_fops;
#endif /* _FO_H */
<file_sep>/kernel/fo_access.c
#include <asm/errno.h>
#include "fo_access.h"
#include "fo_comm.h"
#include "dbg.h"
struct fo_access * fo_acc_start(struct netdev_data *nddata, int pid) {
int err = 0;
struct fo_access *acc = NULL;
if (!nddata) {
printk(KERN_ERR "fo_acc_start: nddata is NULL\n");
return NULL;
}
acc = kzalloc(sizeof(*acc), GFP_KERNEL);
if (!acc) {
printk(KERN_ERR "fo_acc_alloc: failed to allocate fo_access\n");
return NULL;
}
if (nddata->dummy) { /* server doesn't need a queue */
if ((err = kfifo_alloc(&acc->fo_queue,
NETDEV_FO_QUEUE_SIZE,
GFP_KERNEL))) {
printk(KERN_ERR "fo_acc_alloc: failed to allocate fo_queue\n");
goto err;
}
}
init_rwsem(&acc->sem);
acc->access_id = pid;
acc->nddata = nddata;
if (down_write_trylock(&nddata->sem)) {
hash_add(nddata->foacc_htable, &acc->hnode, pid);
ndmgm_get(nddata);
up_write(&acc->nddata->sem);
} else {
printk(KERN_ERR "fo_acc_start: failed to add acc to hashtable\n");
goto err;
}
return acc;
err:
if (nddata->dummy) {
kfifo_free(&acc->fo_queue);
}
kfree(acc);
return NULL;
}
void fo_acc_destroy(
struct fo_access *acc)
{
down_write(&acc->nddata->sem);
hash_del(&acc->hnode);
ndmgm_put(acc->nddata);
up_write(&acc->nddata->sem);
if (acc->nddata->dummy) {
kfifo_free(&acc->fo_queue);
}
kfree(acc);
}
int fo_acc_free_queue(
struct fo_access *acc)
{
int size = 0;
struct fo_req *req = NULL;
down_write(&acc->sem);
/* destroy all requests in the queue */
while (!kfifo_is_empty(&acc->fo_queue)) {
size = kfifo_out(&acc->fo_queue, &req, sizeof(req));
if ( size != sizeof(req) || IS_ERR(req)) {
printk(KERN_ERR "fo_acc_free_queue: failed to fetch from queue, size = %d\n", size);
return 1; /* failure */
}
req->rvalue = -ENODATA;
complete(&req->comp); /* complete all pending file operations */
}
up_write(&acc->sem);
return 0; /* success */
}
/* find the filo operation request with the correct sequence number */
struct fo_req * fo_acc_foreq_find(
struct fo_access *acc,
int seq)
{
/* will hold the first element as a stopper */
struct fo_req *req = NULL;
struct fo_req *tmp = NULL;
int size = 0;
if (down_write_trylock(&acc->sem)) {
while (!kfifo_is_empty(&acc->fo_queue)) {
size = kfifo_out(&acc->fo_queue, &tmp, sizeof(tmp));
if (size < sizeof(tmp) || IS_ERR(tmp)) {
printk(KERN_ERR "fo_acc_foreq_find: failed to get queue element\n");
tmp = NULL;
break;
}
if (req == NULL) {
req = tmp; /* first element */
}
if (tmp->seq == seq) {
break;
}
/* put the wrong element back into the queue */
debug("wrong element, putting it back at the end");
kfifo_in(&acc->fo_queue, &tmp, sizeof(tmp));
if (req == tmp) {
tmp = NULL;
break;
}
}
up_write(&acc->sem);
}
return tmp;
}
int fo_acc_foreq_add(
struct fo_access *acc,
struct fo_req *req)
{
if (down_write_trylock(&acc->sem)) {
kfifo_in(&acc->fo_queue, &req, sizeof(req));
up_write(&acc->sem);
return 0; /* success */
}
return 1; /* failure */
}
<file_sep>/daemon/proxy.c
#include <stdlib.h> /* malloc */
#include <stdio.h> /* printf */
#include <unistd.h> /* close, getpid */
#include <sys/prctl.h> /* prctl */
#include <signal.h> /* signal */
#include <errno.h>
#include "proxy.h"
#include "protocol.h"
#include "signal.h"
#include "helper.h"
#include "netlink.h"
#include "conn.h"
#include "debug.h"
void proxy_setup_signals()
{
/* send SIGHUP when parent process dies */
prctl(PR_SET_PDEATHSIG, SIGHUP);
/* and handle SIGHUP to safely unregister the device */
signal(SIGHUP, proxy_sig_hup);
/* ignore INT since it comes before HUP */
signal(SIGINT, SIG_IGN);
}
int proxy_setup_unixsoc(struct proxy_dev *pdev)
{
if (socketpair(AF_LOCAL, SOCK_STREAM, 0, pdev->us_fd) == -1) {
perror("proxy_setup_unixsoc");
return -1; /* failure */
}
return 0; /* success */
}
struct proxy_dev * proxy_alloc_dev(int connfd)
{
struct proxy_dev *pdev = NULL;
socklen_t addrlen = 0;
pdev = malloc(sizeof(struct proxy_dev));
if (!pdev) {
perror("proxy_alloc_dev(malloc)");
return NULL;
}
pdev->rm_addr = malloc(sizeof(*pdev->rm_addr));
addrlen = sizeof(*pdev->rm_addr);
if (getpeername(connfd, (struct sockaddr *)pdev->rm_addr, &addrlen) == -1) {
perror("proxy_alloc_dev(getperrname)");
return NULL;
}
pdev->rm_fd = connfd;
pdev->pid = getpid();
pdev->client = false; /* this is a server instance */
return pdev;
}
void proxy_destroy(struct proxy_dev *pdev) {
free(pdev->nl_src_addr);
free(pdev->nl_dst_addr);
free(pdev->rm_addr);
free(pdev->us_addr);
free(pdev->rm_ipaddr);
free(pdev->remote_dev_name);
free(pdev->dummy_dev_name);
free(pdev);
}
void proxy_client(struct proxy_dev *pdev)
{
pdev->pid = getpid(); /* make sure you have the right pid */
proxy_setup_signals();
printf("proxy_client: starting connection to server, pid = %d\n", pdev->pid);
if (proxy_setup_unixsoc(pdev) == -1) {
printf("proxy_client: failed to setup control socket\n");
goto err;
}
/* setup a netlink connection with the kernel driver, rest is
* pointless if we can't connect with the kernel driver */
if (netlink_setup(pdev) == -1) {
printf("proxy_client: failed to setup netlink socket!\n");
goto err;
}
/* connect to the server */
if (conn_server(pdev) == -1) {
printf("proxy_client: failed to connect to the server!\n");
goto err;
}
/* send a device registration request to the server */
if (conn_send_dev_reg(pdev) == -1) {
printf("proxy_client: failed to register device on the server!\n");
goto err;
}
/* start loop that will forward all file operations */
if (proxy_loop(pdev) == -1) {
printf("proxy_client: loop broken\n");
}
if (netlink_unregister_dev(pdev) == -1) {
printf("proxy_client: failed to unregister device!\n");
}
err:
proxy_destroy(pdev);
return;
}
void proxy_server(int connfd)
{
struct proxy_dev *pdev = NULL;
proxy_setup_signals();
if ((pdev = proxy_alloc_dev(connfd)) == NULL) {
printf("proxy_server: failed to allocate proxy_dev\n");
return;
}
printf("proxy_server: starting serving client, pid = %d\n", pdev->pid);
if (proxy_setup_unixsoc(pdev) == -1) {
printf("proxy_client: failed to setup control socket\n");
return;
}
/* setup a netlink connection with the kernel driver, rest is pointless
* if we can't connect to the kernel driver */
if (netlink_setup(pdev) == -1) {
printf("proxy_server: failed to setup netlink socket!\n");
return;
}
/* connect to the client */
if (conn_client(pdev) == -1) {
printf("proxy_server: failed to connect to the server!\n");
return;
}
/* receive a device registration request */
if (conn_recv_dev_reg(pdev) == -1) {
printf("proxy_server: failed to register device on the server!\n");
return;
}
/* start loop that will forward all file operations */
if (proxy_loop(pdev) == -1) {
printf("proxy_server: loop broken\n");
}
if (netlink_unregister_dev(pdev) == -1) {
printf("proxy_server: failed to unregister device!\n");
}
proxy_destroy(pdev);
/* close the socket completely */
close(connfd);
return;
}
int proxy_loop(struct proxy_dev *pdev)
{
int maxfd, nready;
fd_set rset;
debug("starting serving connections");
/* Read message from kernel */
while (1) {
FD_ZERO(&rset); /* clear all file descriptors */
FD_SET(pdev->us_fd[0], &rset); /* add unix socket */
FD_SET(pdev->nl_fd, &rset); /* add netlink socket */
FD_SET(pdev->rm_fd, &rset); /* add remote socket */
maxfd = max(pdev->nl_fd, pdev->rm_fd);
maxfd = max(maxfd, pdev->us_fd[0]);
maxfd++; /* this is a count of how far the sockets go */
if ((nready = select(maxfd, /* max number of file descriptors */
&rset, /* read file descriptors */
NULL, /* no write fd */
NULL, /* no exception fd */
NULL) /* no timeout */
) == -1 ) {
perror("proxy_loop(select)");
debug("errno = %d", errno);
return -1; /* failure */
}
if (FD_ISSET(pdev->us_fd[0], &rset)) {
/* message from control unix socket */
if (proxy_control(pdev) == -1) {
return -1;
}
break;
}
if (FD_ISSET(pdev->nl_fd, &rset)) {
if (proxy_handle_netlink(pdev) == -1) {
return -1;
}
}
if (FD_ISSET(pdev->rm_fd, &rset)) {
if (proxy_handle_remote(pdev) == -1) {
return -1;
}
}
}
return 0; /* success */
}
int proxy_control(struct proxy_dev *pdev)
{
printf("proxy_control: not yet implemented\n");
return -1;
}
int proxy_handle_remote(struct proxy_dev *pdev)
{
struct netdev_header *ndhead = NULL;
int rvalue , error;
socklen_t len;
rvalue = getsockopt(pdev->rm_fd, SOL_SOCKET, SO_ERROR, &error, &len);
if (rvalue) {
printf("proxy_handle_remote: connection lost\n");
return -1;
}
ndhead = conn_recv(pdev);
if (!ndhead) {
printf("proxy_handle_remote: failed to receive message\n");
return -1;
}
if (ndhead->msgtype > MSGT_FO_START &&
ndhead->msgtype < MSGT_FO_END) {
/*printf("proxy_handle_remote: FILE OPERATION: %d\n",
ndhead->msgtype);
*/
netlink_send_nlh(pdev, (struct nlmsghdr *)ndhead->payload);
} else {
printf("proxy_handle_remote: unknown message type: %d\n",
ndhead->msgtype);
free(ndhead);
return -1; /* failure */
}
free(ndhead->payload);
free(ndhead);
return 0;
}
/* at the moment the only thing the kernel will send are file
* operations so I will ignore other possibilities */
int proxy_handle_netlink(struct proxy_dev *pdev)
{
struct nlmsghdr *nlh = NULL;
struct netdev_header *ndhead = NULL;
int rvalue = -1;
nlh = netlink_recv(pdev);
if (!nlh) {
printf("proxy_handle_netlink: failed to receive message\n");
goto err;
}
if (nlh->nlmsg_type > MSGT_FO_START &&
nlh->nlmsg_type < MSGT_FO_END) {
/*printf("proxy_handle_netlink: FILE OPERATION: %d\n",
nlh->nlmsg_type);
*/
ndhead = malloc(sizeof(*ndhead));
if (!ndhead) {
perror("proxy_handle_netlink(malloc)");
goto err;
}
ndhead->msgtype = nlh->nlmsg_type;
ndhead->size = nlh->nlmsg_len;
ndhead->payload = nlh;
conn_send(pdev, ndhead);
free(ndhead);
} else {
printf("proxy_handle_netlink: unknown message type: %d\n",
nlh->nlmsg_type);
goto err;
}
/* if error or this message says it wants a response */
rvalue = 0; /* success */
err:
free(nlh);
return rvalue;
}
void proxy_sig_hup(int signo)
{
printf("sig_hup: received signal in process: %d\n", getpid());
/*
if (proxy_unregister_device()) {
printf("sig_hup: failed to send unregister message\n");
}
*/
return;
}
<file_sep>/kernel/fo_access.h
#ifndef _FO_ACCESS_H
#define _FO_ACCESS_H
#include <linux/kfifo.h>
/* fo_access represents one process with one open file descriptor */
struct fo_access {
int access_id; /* identification number of process */
struct netdev_data *nddata; /* netdev device opened by this process */
struct file *filp; /* file descriptor */
struct kfifo fo_queue; /* queue for file operations */
struct rw_semaphore sem; /* necessary since threads can use this */
struct hlist_node hnode; /* to use with hastable */
};
struct fo_access * fo_acc_start(struct netdev_data *nddata, int pid);
void fo_acc_destroy(struct fo_access *acc);
int fo_acc_free_queue(struct fo_access *acc);
struct fo_req * fo_acc_foreq_find(struct fo_access *acc, int seq);
int fo_acc_foreq_add(struct fo_access *acc, struct fo_req *req);
#endif /* _FO_ACCESS_H */
<file_sep>/daemon/netprotocol.h
#ifndef _NETPROTOCL_H
#define _NETPROTOCL_H
struct netdev_header {
int msgtype;
size_t size;
void *payload;
};
#endif
<file_sep>/kernel/fo_comm.h
#ifndef _FO_COMM_H
#define _FO_COMM_H
#include <linux/skbuff.h>
#include <linux/netlink.h> /* for netlink sockets */
#include <linux/fs.h> /* fl_owner_t */
#include <linux/completion.h>
#include "netdevmgm.h"
#include "fo_access.h"
/* necessary since netdevmgm.h and fo_comm.h reference each other */
struct netdev_data;
/* file structure for a queue of operations of dummy device, those will have
* to be allocated fast and from a large pool since there will be a lot */
struct fo_req {
long seq; /* for synchronizing netlink messages */
short msgtype; /* type of file operation */
int access_id; /* identification of opened file */
void *args; /* place for s_fo_OPERATION structures */
void *data; /* place for fo payload */
size_t size; /* size of the s_fo_OPERATION structure */
size_t data_size;
int rvalue; /* informs about success of failure of fo */
struct completion comp; /* completion to release once reply arrives */
};
int fo_send(short msgtype, struct fo_access *acc, void *args, size_t size, void *data, size_t data_size);
int fo_recv(void *data);
int fo_complete(struct fo_access *acc, struct nlmsghdr *nlh, struct sk_buff *skb);
int fo_execute(struct fo_access *acc, struct nlmsghdr *nlh, struct sk_buff *skb);
void * fo_serialize(struct fo_req *req, size_t *bufflen);
struct fo_req * fo_deserialize_toreq(struct fo_req *req, void *data);
struct fo_req * fo_deserialize(struct fo_access *acc, void *data);
#endif /* _FO_COMM_H */
<file_sep>/kernel/fo_send.c
#include "fo_send.h"
#include "fo_comm.h"
#include "fo_struct.h"
#include "protocol.h"
#include "dbg.h"
/* functions for sending and receiving file operations */
loff_t ndfo_send_llseek(struct file *filp, loff_t offset, int whence)
{
loff_t rvalue = 0;
struct s_fo_llseek args = {
.offset = offset,
.whence = whence,
.rvalue = -EIO
};
rvalue = fo_send(MSGT_FO_LLSEEK,
filp->private_data,
&args, sizeof(args),
NULL, 0);
if (rvalue < 0) {
return rvalue;
}
return args.rvalue;
}
ssize_t ndfo_send_read(struct file *filp, char __user *data, size_t size, loff_t *offset)
{
ssize_t rvalue = 0;
struct s_fo_read args = {
.size = size,
.offset = *offset,
.rvalue = -EIO
};
if (size >= NETDEV_MESSAGE_LIMIT) {
printk(KERN_ERR "ndfo_send_read: buffor too big for message\n");
return -EINVAL;
}
args.data = kmalloc(size, GFP_KERNEL);
if (!args.data) {
printk(KERN_ERR "ndfo_send_read: failed to allocate args.data\n");
return -ENOMEM;
}
rvalue = fo_send(MSGT_FO_READ,
filp->private_data,
&args, sizeof(args),
args.data, 0);
if (rvalue < 0) {
return rvalue;
}
*offset = args.offset;
rvalue = copy_to_user(data, args.data, args.rvalue);
if (rvalue > 0) {
printk(KERN_ERR "ndfo_send_read: failed to copy to user\n");
return -1;
}
kfree(args.data);
return args.rvalue;
}
ssize_t ndfo_send_write(struct file *filp, const char __user *data, size_t size, loff_t *offset)
{
size_t rvalue = 0;
struct s_fo_write args = {
.size = size,
.offset = *offset,
.rvalue = -EIO
};
if (size >= NETDEV_MESSAGE_LIMIT) {
printk(KERN_ERR "ndfo_send_write: buffor too big for message\n");
return -EINVAL;
}
args.data = kmalloc(size, GFP_KERNEL);
if (!args.data) {
printk(KERN_ERR "ndfo_send_write: failed to allocate args.data\n");
return -ENOMEM;
}
*offset = args.offset;
rvalue = copy_from_user(args.data, data, size);
if (rvalue > 0) {
printk(KERN_ERR "ndfo_send_write: failed to copy from user\n");
return -EIO;
}
rvalue = fo_send(MSGT_FO_WRITE,
filp->private_data,
&args, sizeof(args),
args.data, size);
if (rvalue < 0) {
return rvalue;
}
kfree(args.data);
return args.rvalue;
}
size_t ndfo_send_aio_read(struct kiocb *a, const struct iovec *b, unsigned long c, loff_t offset)
{
return -EIO;
}
ssize_t ndfo_send_aio_write(struct kiocb *a, const struct iovec *b, unsigned long c, loff_t d)
{
return -EIO;
}
unsigned int ndfo_send_poll(struct file *filp, struct poll_table_struct *wait)
{
return -EIO;
}
long ndfo_send_unlocked_ioctl(struct file *filp, unsigned int cmd, unsigned long arg)
{
long rvalue = 0;
struct s_fo_unlocked_ioctl args = {
.cmd = cmd,
.arg = arg,
.rvalue = -EIO
};
rvalue = fo_send(MSGT_FO_UNLOCKED_IOCTL,
filp->private_data,
&args, sizeof(args),
NULL, 0);
if (rvalue < 0) {
return rvalue;
}
return args.rvalue;
}
long ndfo_send_compat_ioctl(struct file *filp, unsigned int cmd, unsigned long arg)
{
long rvalue = 0;
struct s_fo_compat_ioctl args = {
.cmd = cmd,
.arg = arg,
.rvalue = -EIO
};
rvalue = fo_send(MSGT_FO_COMPAT_IOCTL,
filp->private_data,
&args, sizeof(args),
NULL, 0);
if (rvalue < 0) {
return rvalue;
}
return args.rvalue;
}
int ndfo_send_mmap(struct file *filp, struct vm_area_struct *b)
{
return -EIO;
}
int ndfo_send_open(struct inode *inode, struct file *filp)
{
int rvalue = 0;
struct netdev_data *nddata = NULL;
struct fo_access *acc = NULL;
struct s_fo_open args = {
.rvalue = -EIO
};
nddata = ndmgm_find(ndmgm_find_pid(inode->i_cdev->dev));
/* get the device connected with this file */
acc = fo_acc_start(nddata, current->pid);
if (!acc) {
printk(KERN_ERR "ndfo_send_open: failed to allocate acc\n");
return -ENOMEM;
}
/* set private data for easy access to netdev_data struct */
filp->private_data = (void*)acc;
rvalue = fo_send(MSGT_FO_OPEN,
filp->private_data,
&args, sizeof(args),
NULL, 0);
if (rvalue < 0) {
return rvalue;
}
return args.rvalue;
}
int ndfo_send_flush(struct file *filp, fl_owner_t id)
{
int rvalue = 0;
struct s_fo_flush args = {
.rvalue = -EIO
};
rvalue = fo_send(MSGT_FO_FLUSH,
filp->private_data,
&args, sizeof(args),
NULL, 0);
if (rvalue < 0) {
return rvalue;
}
return args.rvalue;
}
int ndfo_send_release(struct inode *a, struct file *filp)
{
int rvalue = 0;
struct s_fo_release args = {
.rvalue = -EIO
};
rvalue = fo_send(MSGT_FO_RELEASE,
filp->private_data,
&args, sizeof(args),
NULL, 0);
if (rvalue < 0) {
return rvalue;
}
return args.rvalue;
}
int ndfo_send_fsync(struct file *filp, loff_t b, loff_t offset, int d)
{
return -EIO;
}
int ndfo_send_aio_fsync(struct kiocb *a, int b)
{
return -EIO;
}
int ndfo_send_fasync(int a, struct file *filp, int c)
{
return -EIO;
}
int ndfo_send_lock(struct file *filp, int b, struct file_lock *c)
{
return -EIO;
}
ssize_t ndfo_send_sendpage(struct file *filp, struct page *b, int c, size_t d, loff_t *offset, int f)
{
return -EIO;
}
unsigned long ndfo_send_get_unmapped_area(struct file *filp, unsigned long b, unsigned long c,unsigned long d, unsigned long e)
{
return -EIO;
}
int ndfo_send_check_flags(int a)
{
return -EIO;
}
int ndfo_send_flock(struct file *filp, int b, struct file_lock *c)
{
return -EIO;
}
ssize_t ndfo_send_splice_write(struct pipe_inode_info *a, struct file *filp, loff_t *offset, size_t d, unsigned int e)
{
return -EIO;
}
ssize_t ndfo_send_splice_read(struct file *filp, loff_t *offset, struct pipe_inode_info *c, size_t d, unsigned int e)
{
return -EIO;
}
int ndfo_send_setlease(struct file *filp, long b, struct file_lock **c)
{
return -EIO;
}
long ndfo_send_fallocate(struct file *filp, int b, loff_t offset, loff_t len)
{
return -EIO;
}
int ndfo_send_show_fdinfo(struct seq_file *a, struct file *filp)
{
return -EIO;
}
<file_sep>/kernel/netdevmgm.c
#include <linux/slab.h> /* kmalloc, kzalloc, kfree and so on */
#include <linux/device.h>
#include "netdevmgm.h"
#include "fo.h"
#include "dbg.h"
static DEFINE_HASHTABLE(netdev_htable, NETDEV_HTABLE_DEV_SIZE);
static struct rw_semaphore netdev_htable_sem;
static struct rw_semaphore netdev_minor_sem;
static int netdev_max_minor;
static int *netdev_minors_used; /* array with pids to all the devices */
struct netdev_data * ndmgm_alloc_data(
int nlpid,
char *name,
int minor)
{
struct netdev_data *nddata;
char pool_name[30];
sprintf(pool_name, "%s:%s%d", NETDEV_REQ_POOL_NAME, name, minor);
nddata = kzalloc(sizeof(*nddata), GFP_KERNEL);
if (!nddata) {
printk(KERN_ERR "ndmgm_alloc_data: failed to allocate nddata\n");
return NULL;
}
nddata->devname = kzalloc(strlen(name)+1, GFP_KERNEL);
if (!nddata->devname) {
printk(KERN_ERR "ndmgm_alloc_data: failed to allocate nddata\n");
goto free_devname;
}
nddata->cdev = kzalloc(sizeof(*nddata->cdev), GFP_KERNEL);
if (!nddata->cdev) {
printk(KERN_ERR "ndmgm_alloc_data: failed to allocate nddata->cdev\n");
goto free_nddata;
}
nddata->queue_pool = kmem_cache_create(pool_name,
sizeof(struct fo_req),
/* no alignment, flags or constructor */ 0, 0, NULL);
if (!nddata->queue_pool) {
printk(KERN_ERR "ndmgm_alloc_data: failed to allocate queue_pool\n");
goto free_cdev;
}
sprintf(nddata->devname, "/dev/%s", name);
hash_init(nddata->foacc_htable);
init_rwsem(&nddata->sem);
spin_lock_init(&nddata->nllock);
atomic_set(&nddata->curseq, 0);
nddata->nlpid = nlpid;
nddata->active = true;
return nddata;
free_cdev:
kfree(nddata->cdev);
free_devname:
kfree(nddata->devname);
free_nddata:
kfree(nddata);
return NULL;
}
int ndmgm_create_dummy(
int nlpid,
char *name)
{
int err = 0;
int minor = -1;
struct netdev_data *nddata = NULL;
minor = ndmgm_get_minor(nlpid);
if (minor == -1) {
printk(KERN_ERR "ndmgm_create_dummy: could not get minor\n");
return 1;
}
debug("creating dummy device: /dev/%s", name);
if ( (nddata = ndmgm_alloc_data(nlpid, name, minor)) == NULL ) {
printk(KERN_ERR "ndmgm_create_dummy: failed to create netdev_data\n");
return 1;
}
cdev_init(nddata->cdev, &netdev_fops);
nddata->cdev->owner = THIS_MODULE;
nddata->cdev->dev = MKDEV(MAJOR(netdev_devno), minor);
nddata->dummy = true; /* should be true for a dummy device */
/* tell the kernel the cdev structure is ready,
* if it is not do not call cdev_add */
err = cdev_add(nddata->cdev, nddata->cdev->dev, 1);
/* Unlikely but might fail */
if (unlikely(err)) {
printk(KERN_ERR "Error %d adding netdev\n", err);
goto free_nddata;
}
nddata->device = device_create(netdev_class,
NULL, /* no aprent device */
nddata->cdev->dev, /* major and minor */
nddata, /* device data for callback */
"%s", /* defines name of the device */
name);
if (IS_ERR(nddata->device)) {
err = PTR_ERR(nddata->device);
printk(KERN_WARNING "[target] Error %d while trying to name %s\n",
err, name);
goto undo_cdev;
}
/* add the device to hashtable with all devices since it's ready */
ndmgm_get(nddata); /* increase count for hashtable */
hash_add(netdev_htable, &nddata->hnode, (int)nlpid);
return 0; /* success */
undo_cdev:
cdev_del(nddata->cdev);
free_nddata:
ndmgm_free_data(nddata);
return err;
}
int ndmgm_create_server(
int nlpid,
char *name)
{
struct netdev_data *nddata = NULL;
char pool_name[30];
sprintf(pool_name, "%s:%s", NETDEV_REQ_POOL_NAME, name);
debug("creating server for: %s", name);
nddata = kzalloc(sizeof(*nddata), GFP_KERNEL);
if (!nddata) {
printk(KERN_ERR "ndmgm_create_server: failed to allocate nddata\n");
return 1;
}
nddata->devname = kzalloc(strlen(name)+1, GFP_KERNEL);
if (!nddata->devname) {
printk(KERN_ERR "ndmgm_create_server: failed to allocate nddata\n");
goto free_nddata;
}
nddata->queue_pool = kmem_cache_create(pool_name,
sizeof(struct fo_req),
/* no alignment, flags or constructor */ 0, 0, NULL);
if (!nddata->queue_pool) {
printk(KERN_ERR "ndmgm_alloc_data: failed to allocate queue_pool\n");
goto free_devname;
}
memcpy(nddata->devname, name, strlen(name)+1);
init_rwsem(&nddata->sem);
spin_lock_init(&nddata->nllock);
atomic_set(&nddata->curseq, 0);
nddata->nlpid = nlpid;
nddata->active = true;
nddata->dummy = false; /* should be false for a server device */
/* add the device to hashtable with all devices since it's ready */
ndmgm_get(nddata); /* increase count for hashtable */
hash_add(netdev_htable, &nddata->hnode, (int)nlpid);
/* TODO find cdev and device of the dev we are serving */
return 0; /* success */
free_devname:
kfree(nddata->devname);
free_nddata:
kfree(nddata);
return 1;
}
void ndmgm_free_data(
struct netdev_data *nddata)
{
kmem_cache_destroy(nddata->queue_pool);
kfree(nddata->cdev);
kfree(nddata->devname);
kfree(nddata);
}
/* returns netdev_data based on pid, you have to make sure to
* increment the reference counter */
struct netdev_data* ndmgm_find(int nlpid)
{
struct netdev_data *nddata = NULL;
if (nlpid == -1) {
printk("ndmgm_find: invalid pid\n");
return NULL;
}
if (down_read_trylock(&netdev_htable_sem)) {
hash_for_each_possible(netdev_htable, nddata, hnode, (int)nlpid) {
if ( nddata->nlpid == nlpid ) {
up_read(&netdev_htable_sem);
return nddata;
}
}
up_read(&netdev_htable_sem);
}
return NULL;
}
struct fo_access * ndmgm_find_acc(
struct netdev_data *nddata,
int access_id)
{
struct fo_access *acc = NULL;
if (down_read_trylock(&nddata->sem)) {
hash_for_each_possible(nddata->foacc_htable,
acc,
hnode,
access_id) {
if ( acc->access_id == access_id ) {
up_read(&nddata->sem);
return acc;
}
}
up_read(&nddata->sem);
}
return NULL;
}
void ndmgm_put_minor(
int minor)
{
int i = 0;
if (down_write_trylock(&netdev_minor_sem)) {
netdev_minors_used[minor] = -1;
if (minor == netdev_max_minor) {
for (i = minor; i > 0; i--) {
if (netdev_minors_used[i] != -1) {
netdev_max_minor = i;
}
}
}
up_write(&netdev_minor_sem);
}
}
int ndmgm_get_minor(
int pid)
{
int minor = -1;
int i = 0;
if (down_write_trylock(&netdev_minor_sem)) {
if (netdev_max_minor == NETDEV_MAX_DEVICES) {
printk("ndmgm_get_minor: device limit reached: %d\n",
NETDEV_MAX_DEVICES);
up_read(&netdev_minor_sem);
return -1;
}
for (i = 0; i <= netdev_max_minor+1; i++) {
if (netdev_minors_used[i] == -1) {
netdev_minors_used[i] = pid;
minor = i;
break;
}
}
if (minor >= 0) {
netdev_max_minor = max(netdev_max_minor, minor);
}
up_write(&netdev_minor_sem);
}
return minor;
}
int ndmgm_find_pid(
dev_t dev)
{
int pid = -1;
if (down_read_trylock(&netdev_minor_sem)) {
pid = netdev_minors_used[MINOR(dev)];
up_read(&netdev_minor_sem);
}
return pid;
}
/* this function safely increases the current sequence number */
int ndmgm_incseq(
struct netdev_data *nddata)
{
int rvalue;
atomic_inc(&nddata->curseq);
rvalue = atomic_read(&nddata->curseq);
return rvalue;
}
int ndmgm_find_destroy(
int nlpid)
{
struct netdev_data *nddata = NULL;
nddata = ndmgm_find(nlpid);
if (!nddata) {
printk(KERN_ERR "ndmgm_find_destroy: no such device\n");
return 1; /* failure */
}
if (down_read_trylock(&netdev_htable_sem)) {
hash_del(&nddata->hnode);
ndmgm_put(nddata);
up_read(&netdev_htable_sem);
}
return ndmgm_destroy(nddata);
}
int ndmgm_destroy(
struct netdev_data *nddata)
{
int rvalue;
if (!nddata) {
printk(KERN_ERR "ndmgm_destroy: nddata is NULL\n");
return 1; /* failure */
}
debug("destroying device %s", nddata->devname);
if (nddata->dummy == true) {
rvalue = ndmgm_destroy_dummy(nddata);
} else if (nddata->dummy == false) {
rvalue = ndmgm_destroy_server(nddata);
}
return rvalue;
}
int ndmgm_destroy_allacc(
struct netdev_data *nddata)
{
struct fo_access *acc = NULL;
struct hlist_node *tmp;
int i = 0;
if (down_write_trylock(&netdev_htable_sem)) {
hash_for_each_safe(nddata->foacc_htable, i, tmp, acc, hnode) {
hash_del(&nddata->hnode); /* delete the element from table */
/* make sure all pending operations are completed */
if (fo_acc_free_queue(acc)) {
printk(KERN_ERR "ndmgm_destroy_allacc: failed to free queue\n");
return 1; /* failure */
}
fo_acc_destroy(acc);
}
up_write(&netdev_htable_sem);
return 0; /* success */
}
return 1; /* failure */
}
int ndmgm_destroy_dummy(
struct netdev_data *nddata)
{
if (down_write_trylock(&nddata->sem)) {
nddata->active = false;
if (ndmgm_destroy_allacc(nddata)) {
printk(KERN_ERR
"ndmgm_destroy_dummy: failed to stop all acc\n");
up_write(&nddata->sem);
return 1; /* failure */
}
/* should never happen but better test for it */
if (ndmgm_refs(nddata) > 1) {
printk(KERN_ERR
"ndmgm_destroy_dummy: more than one ref left: %d\n",
ndmgm_refs(nddata));
up_write(&nddata->sem);
return 1; /* failure */
}
device_destroy(netdev_class, nddata->cdev->dev);
cdev_del(nddata->cdev);
up_write(&nddata->sem); /* has to be unlocked before kfree */
ndmgm_put_minor(MINOR(nddata->cdev->dev));
ndmgm_free_data(nddata); /* finally free netdev_data */
return 0; /* success */
}
debug("failed to destroy netdev_data");
return 1; /* failure */
}
int ndmgm_destroy_server(
struct netdev_data *nddata)
{
if (down_write_trylock(&nddata->sem)) {
nddata->active = false;
if (ndmgm_refs(nddata) > 1) {
printk(KERN_ERR
"ndmgm_destroy_server: more than one ref left: %d\n",
ndmgm_refs(nddata));
up_write(&nddata->sem); /* has to be unlocked before kfree */
return 1; /* failure */
}
up_write(&nddata->sem); /* has to be unlocked before kfree */
kmem_cache_destroy(nddata->queue_pool);
kfree(nddata->devname);
kfree(nddata);
return 0; /* success */
}
debug("failed to destroy netdev_data");
return 1; /* failure */
}
int ndmgm_end(void)
{
int i = 0;
struct netdev_data *nddata = NULL;
struct hlist_node *tmp;
debug("cleaning devices");
if (down_write_trylock(&netdev_htable_sem)) {
hash_for_each_safe(netdev_htable, i, tmp, nddata, hnode) {
debug("deleting dev pid = %d", nddata->nlpid);
hash_del(&nddata->hnode); /* delete the element from table */
ndmgm_put(nddata);
if (ndmgm_destroy(nddata)) {
printk(KERN_ERR "netdev_end: failed to destroy nddata\n");
}
}
kfree(netdev_minors_used);
up_write(&netdev_htable_sem);
return 0; /* success */
}
debug("failed to unlock hashtable");
return 1; /* failure */
}
void ndmgm_prepare(void)
{
int i;
/* create and array for all drivices which will be indexed with
* minor numbers of those devices */
netdev_minors_used = kcalloc(NETDEV_MAX_DEVICES,
sizeof(*netdev_minors_used),
GFP_KERNEL);
for (i = 0; i < NETDEV_MAX_DEVICES; i++) {
netdev_minors_used[i] = -1; /* -1 means free minor number */
}
netdev_max_minor = 0;
/* create the hashtable which will store data about created devices
* and for easy access through pid */
hash_init(netdev_htable);
}
void ndmgm_get(
struct netdev_data *nddata)
{
//debug("dev: %15s, from: %pS", nddata->devname, __builtin_return_address(0));
atomic_inc(&nddata->users);
}
void ndmgm_put(
struct netdev_data *nddata)
{
//debug("dev: %15s, from: %pS", nddata->devname, __builtin_return_address(0));
atomic_dec(&nddata->users);
}
int ndmgm_refs(
struct netdev_data *nddata)
{
return atomic_read(&nddata->users);
}
<file_sep>/kernel/netdev.h
#ifndef _NETDEV_H
#define _NETDEV_H
/* this will be provided by the name variable in netdev_data struct */
#define NETDEV_NAME "netdev"
/* let the kernel decide the major number */
#define NETDEV_MAJOR 0
/* defines the number which is the power of two which gives the max number
* of devices this driver will manage and store in the hashtable */
#define NETDEV_HTABLE_DEV_SIZE 5
#define NETDEV_HTABLE_ACC_SIZE 5
#define NETDEV_MAX_DEVICES 32 /* 2 to the power of 5(HTABLE_DEV_SIZE)*/
#define NETDEV_MAX_OPEN_FILES 32 /* 2 to the power of 5(HTABLE_ACC_SIZE) */
#define NETDEV_FO_QUEUE_SIZE 64 /* has to be a power of 2 */
#define NETDEV_REQ_POOL_NAME "netdev_req_pool" /* for debugging */
#endif /* _NETDEV_H */
<file_sep>/kernel/fo_comm.c
#include <linux/slab.h>
#include <net/netlink.h>
#include <asm/uaccess.h>
#include "protocol.h"
#include "netlink.h"
#include "fo_recv.h"
#include "fo_access.h"
#include "dbg.h"
static void pk(void) {
printk(KERN_DEBUG "netdev: file operation from:\"%s\", PID: %d - %pS\n",
current->comm,
current->pid,
__builtin_return_address(0));
}
int fo_send(
short msgtype,
struct fo_access *acc,
void *args,
size_t size,
void *data,
size_t data_size)
{
int rvalue = 0;
size_t bufflen = 0;
void *buffer = NULL;
struct fo_req *req = NULL;
//pk(); /* for debugging only */
if (!acc->nddata->active) {
return -EBUSY;
}
/* increment reference counter of netdev_data */
ndmgm_get(acc->nddata);
req = kmem_cache_alloc(acc->nddata->queue_pool, GFP_KERNEL);
if (!req) {
printk(KERN_ERR "senf_fo: failed to allocate queue_pool\n");
rvalue = -ENOMEM;
goto out;
}
req->rvalue = -1; /* assume failure, server has to change it to 0 */
req->msgtype = msgtype;
req->access_id = acc->access_id;
req->args = args;
req->size = size;
req->data = data;
req->data_size = data_size;
init_completion(&req->comp);
/* add the req to a queue of requests */
fo_acc_foreq_add(acc, req);
buffer = fo_serialize(req, &bufflen);
if (!buffer) {
printk(KERN_ERR "fo_send: failed to serialize req\n");
rvalue = -ENODATA;
goto out;
}
if (!(req->seq = ndmgm_incseq(acc->nddata))) {
printk(KERN_ERR "fo_send: failed to increment curseq\n");
rvalue = -EBUSY;
goto out;
}
/* send the file operation request */
rvalue = netlink_send(acc->nddata,
req->seq,
req->msgtype,
NLM_F_REQUEST,
buffer,
bufflen);
if (rvalue < 0) {
printk(KERN_ERR "fo_send: failed to send file operation\n");
rvalue = -ECANCELED;
goto out;
}
/* wait for completion, it will be signaled once a reply is received */
wait_for_completion(&req->comp);
rvalue = req->rvalue;
out:
kfree(buffer);
ndmgm_put(acc->nddata);
kmem_cache_free(acc->nddata->queue_pool, req);
if (msgtype == MSGT_FO_RELEASE) {
fo_acc_destroy(acc);
}
return rvalue;
}
/* this function will be executed as a new thread with kthread_run */
int fo_recv(
void *data)
{
struct sk_buff *skb = data;
struct netdev_data *nddata = NULL;
struct fo_access *acc = NULL;
struct nlmsghdr *nlh = NULL;
int msgtype = 0;
int rvalue = 0; /* success */
int pid = 0;
nlh = nlmsg_hdr(skb);
msgtype = nlh->nlmsg_type;
nddata = ndmgm_find(nlh->nlmsg_pid);
if (IS_ERR(nddata)) {
printk(KERN_ERR "fo_recv: failed to find device for pid = %d\n",
nlh->nlmsg_pid);
do_exit(-ENODEV); /* failure */
}
ndmgm_get(nddata);
memcpy(&pid, NLMSG_DATA(nlh), sizeof(pid));
acc = ndmgm_find_acc(nddata, pid);
if (!acc) {
if (msgtype != MSGT_FO_OPEN) {
printk(KERN_ERR "fo_recv: no opened file for pid = %d\n", pid);
do_exit(-ENFILE);
}
acc = fo_acc_start(nddata, pid);
if (!acc) {
printk(KERN_ERR "fo_recv: failed to allocate acc\n");
do_exit(-ENOMEM);
}
}
if (nddata->dummy) {
rvalue = fo_complete(acc, nlh, skb);
} else {
rvalue = fo_execute(acc, nlh, skb);
}
/* if open failed we need to free acc */
if (rvalue == -1 && msgtype == MSGT_FO_OPEN) {
fo_acc_destroy(acc);
}
ndmgm_put(nddata);
do_exit(rvalue);
}
int fo_complete(
struct fo_access *acc,
struct nlmsghdr *nlh,
struct sk_buff *skb)
{
struct fo_req *req = NULL;
//debug("completing, pid = %d, seq = %d", acc->access_id, nlh->nlmsg_seq);
/* first element in paylod is access ID */
req = fo_acc_foreq_find(acc, nlh->nlmsg_seq);
if (!req) {
printk(KERN_ERR "fo_complete: failed to obtain fo request\n");
return -1;
}
if (!fo_deserialize_toreq(req, NLMSG_DATA(nlh))) {
printk(KERN_ERR "fo_complete: failed to deserialize req\n");
return -1;
}
complete(&req->comp);
dev_kfree_skb(skb); /* fo_execute frees skb by sending unicat */
return 0;
}
int fo_execute(
struct fo_access *acc,
struct nlmsghdr *nlh,
struct sk_buff *skb)
{
int (*fofun)(struct fo_access*, struct fo_req*) = NULL;
struct sk_buff *skbtmp = NULL;
struct fo_req *req = NULL;
void *buff = NULL;
size_t bufflen = 0;
int fonum = 0;
int rvalue = -ENODATA;
int msgtype = nlh->nlmsg_type;
req = fo_deserialize(acc, NLMSG_DATA(nlh));
if (!req) {
printk(KERN_ERR "fo_execute: failed to deserialize req\n");
goto err;
}
//debug("executing, pid = %d, seq = %d", acc->access_id, nlh->nlmsg_seq);
/* get number of file operation for array */
fonum = msgtype - (MSGT_FO_START+1);
/* get the correct file operation function from the array */
fofun = netdev_recv_fops[fonum];
if (!fofun) {
printk(KERN_ERR "fo_execute: file operation not implemented\n");
goto err;
}
/* execute the file operation */
req->rvalue = fofun(acc, req);
if (req->rvalue == -1) {
printk(KERN_ERR "fo_execute: file operation failed = %d\n", msgtype);
goto err;
}
/* send back the result to the server in userspace */
buff = fo_serialize(req, &bufflen);
if (!buff) {
printk(KERN_ERR "fo_execute: failed to serialize req\n");
goto err;
}
if (bufflen == nlmsg_len(nlh)) {
memcpy(nlmsg_data(nlh), buff, bufflen);
} else {
/* expand skb and put in data, possibly split into parts */
skbtmp = netlink_pack_skb(nlh, buff, bufflen);
if (!skb) {
printk(KERN_ERR "fo_execute: failed to put data into skb\n");
goto err;
}
dev_kfree_skb(skb);
skb = skbtmp;
}
rvalue = 0; /* success */
err:
/* rvalue is -1 so sending it back untouched will mean failure */
netlink_send_skb(acc->nddata, skb);
kfree(req->args);
kfree(req->data);
kmem_cache_free(acc->nddata->queue_pool, req);
kfree(buff);
if (msgtype == MSGT_FO_RELEASE) {
fo_acc_destroy(acc);
}
return rvalue;
}
/* file operation structure:
* 0 - int - access ID (HAS TO BE FIRST)
* 1 - int - return value of operation
* 2 - size_t - size of args structure
* 3 - size_t - size of data/payload
* 4 - args - struct with fo args
* 5 - data - payload
*/
void * fo_serialize(
struct fo_req *req,
size_t *bufflen)
{
void *data = NULL;
size_t size = 0;
*bufflen = sizeof(req->access_id) +
sizeof(req->rvalue) +
sizeof(req->size) +
sizeof(req->data_size) +
req->size +
req->data_size;
data = kzalloc(*bufflen, GFP_KERNEL);
if (!data) {
printk(KERN_ERR "fo_serialize: failed to allocate data\n");
return NULL; /* failure */
}
memcpy(data + size, &req->access_id, sizeof(req->access_id));
size += sizeof(req->access_id);
memcpy(data + size, &req->rvalue, sizeof(req->rvalue));
size += sizeof(req->rvalue);
memcpy(data + size, &req->size, sizeof(req->size));
size += sizeof(req->size);
memcpy(data + size, &req->data_size, sizeof(req->data_size));
size += sizeof(req->data_size);
if (req->args == NULL) {
return data;
}
memcpy(data + size, req->args, req->size);
size += req->size;
if (req->data_size == 0) {
return data;
}
memcpy(data + size, req->data, req->data_size);
return data;
}
struct fo_req * fo_deserialize_toreq(
struct fo_req *req,
void *data)
{
size_t size = 0;
if (!req) {
printk(KERN_ERR "fo_deserialize_toreq: req is NULL\n");
return NULL; /* failure */
}
/* get all the data */
memcpy(&req->access_id, data + size, sizeof(req->access_id));
size += sizeof(req->access_id);
memcpy(&req->rvalue, data + size, sizeof(req->rvalue));
size += sizeof(req->rvalue);
memcpy(&req->size, data + size, sizeof(req->size));
size += sizeof(req->size);
memcpy(&req->data_size, data + size, sizeof(req->data_size));
if (req->size == 0) {
return req;
}
size += sizeof(req->data_size);
if (!req->args) {
req->args = kzalloc(req->size, GFP_KERNEL);
if (!req->args) {
printk(KERN_ERR "fo_deserialize_toreq: failed to allocate args\n");
return NULL;
}
}
memcpy(req->args, data + size, req->size);
if (req->data_size == 0) {
return req;
}
size += req->size;
if (!req->data) {
req->data = kzalloc(req->data_size, GFP_KERNEL);
if (!req->data) {
printk(KERN_ERR "fo_deserialize: failed to allocate data\n");
return NULL;
}
}
memcpy(req->data, data + size, req->data_size);
return req;
}
struct fo_req * fo_deserialize(
struct fo_access *acc,
void *data)
{
struct fo_req *req = NULL;
size_t size = 0;
req = kmem_cache_alloc(acc->nddata->queue_pool, GFP_KERNEL);
if (!req) {
printk(KERN_ERR "fo_deserialize: failed to allocate req\n");
return NULL; /* failure */
}
req->args = NULL;
req->data = NULL;
/* get all the data */
memcpy(&req->access_id, data + size, sizeof(req->access_id));
size += sizeof(req->access_id);
memcpy(&req->rvalue, data + size, sizeof(req->rvalue));
size += sizeof(req->rvalue);
memcpy(&req->size, data + size, sizeof(req->size));
size += sizeof(req->size);
memcpy(&req->data_size, data + size, sizeof(req->data_size));
if (req->size == 0) {
return req;
}
size += sizeof(req->data_size);
req->args = kzalloc(req->size, GFP_KERNEL);
if (!req->args) {
printk(KERN_ERR "fo_deserialize: failed to allocate args\n");
goto free_req;
}
memcpy(req->args, data + size, req->size);
if (req->data_size == 0) {
return req;
}
size += req->size;
req->data = kzalloc(req->data_size, GFP_KERNEL);
if (!req->data) {
printk(KERN_ERR "fo_deserialize: failed to allocate data\n");
goto free_req;
}
memcpy(req->data, data + size, req->data_size);
return req;
free_req:
kfree(req->data);
kfree(req->args);
kfree(req);
return NULL;
}
<file_sep>/kernel/fo_recv.c
#include <linux/fs.h>
#include <linux/fcntl.h>
#include "fo_recv.h"
#include "fo_struct.h"
#include "fo_access.h"
#include "dbg.h"
int ndfo_recv_llseek(struct fo_access *acc, struct fo_req *req) {
struct s_fo_llseek *args = req->args;
if (!acc->filp->f_op->llseek) {
printk(KERN_ERR "ndfo_recv_llseek: operation is NULL\n");
return -1;
}
args->rvalue = acc->filp->f_op->llseek(acc->filp,
args->offset,
args->whence);
return 0;
}
int ndfo_recv_read(struct fo_access *acc, struct fo_req *req) {
struct s_fo_read *args = req->args;
req->data = kzalloc(args->size , GFP_KERNEL);
req->data_size = args->size;
args->rvalue = acc->filp->f_op->read(acc->filp,
req->data,
args->size,
&args->offset);
return 0;
}
int ndfo_recv_write(struct fo_access *acc, struct fo_req *req) {
struct s_fo_write *args = req->args;
args->rvalue = acc->filp->f_op->write(acc->filp,
req->data,
args->size,
&args->offset);
/* don't send written data back */
kfree(req->data);
req->data = NULL;
req->data_size = 0;
return 0;
}
int ndfo_recv_poll(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int ndfo_recv_unlocked_ioctl(struct fo_access *acc, struct fo_req *req) {
struct s_fo_unlocked_ioctl *args = req->args;
args->rvalue = acc->filp->f_op->unlocked_ioctl(acc->filp,
args->cmd,
args->arg);
return -1;
}
int ndfo_recv_compat_ioctl(struct fo_access *acc, struct fo_req *req) {
struct s_fo_compat_ioctl *args = req->args;
args->rvalue = acc->filp->f_op->compat_ioctl(acc->filp,
args->cmd,
args->arg);
return 0;
}
int ndfo_recv_mmap(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int ndfo_recv_open(struct fo_access *acc, struct fo_req *req) {
struct s_fo_open *args = req->args;
int err = 0;
int flags = O_RDWR | O_LARGEFILE;
int mode = 0;
acc->filp = filp_open(acc->nddata->devname, flags, mode);
if (IS_ERR(acc->filp)) {
err = PTR_ERR(acc->filp);
printk(KERN_ERR "ndfo_recv_open_req: err = %d\n", err);
return -1; /* failure */
}
if (!acc->filp->f_op) {
printk(KERN_ERR "ndfo_recv_open_req: no file operations\n");
return -1; /* failure */
}
args->rvalue = 0;
return 0; /* success */
}
int ndfo_recv_flush(struct fo_access *acc, struct fo_req *req) {
struct s_fo_flush *args = req->args;
if (!acc->filp->f_op->flush) {
printk(KERN_ERR "ndfo_recv_flush: operation is NULL\n");
return -1;
}
args->rvalue = acc->filp->f_op->flush(acc->filp, NULL);
return 0;
}
int ndfo_recv_release(struct fo_access *acc, struct fo_req *req) {
struct s_fo_release *args = req->args;
args->rvalue = filp_close(acc->filp, NULL);
return 0;
}
int ndfo_recv_fsync(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int ndfo_recv_aio_fsync(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int ndfo_recv_fasync(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int ndfo_recv_lock(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int ndfo_recv_check_flags(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int ndfo_recv_flock(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int ndfo_recv_splice_write(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int ndfo_recv_splice_read(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int ndfo_recv_setlease(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int ndfo_recv_fallocate(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int ndfo_recv_show_fdinfo(struct fo_access *acc, struct fo_req *req) {
return -1;
}
int (*netdev_recv_fops[])(struct fo_access *, struct fo_req*) = {
ndfo_recv_llseek,
ndfo_recv_read,
ndfo_recv_write,
NULL, /* will be hard to implement because of kiocb */
NULL, /* will be hard to implement because of kiocb */
NULL, /* useless for devices */
ndfo_recv_poll,/* NULL assumes it's not blocing */
ndfo_recv_unlocked_ioctl,
ndfo_recv_compat_ioctl,
NULL, /* for mapping device memory to user space */
ndfo_recv_open,
ndfo_recv_flush, /* rarely used for devices */
ndfo_recv_release,
ndfo_recv_fsync,
NULL, /* will be hard to implement because of kiocb */
ndfo_recv_fasync,
NULL, /* almost never implemented */
NULL, /* usually not used, for sockets */
NULL, /* mostly NULL */
ndfo_recv_check_flags, /* fcntl(F_SETFL...) */
ndfo_recv_flock,
NULL, /* needs DMA */
NULL, /* needs DMA */
ndfo_recv_setlease,
ndfo_recv_fallocate,
ndfo_recv_show_fdinfo
};
<file_sep>/daemon/netlink.c
#include <stdio.h> /* perror */
#include <stdlib.h> /* malloc */
#include "netlink.h"
#include "protocol.h"
#include "netprotocol.h"
#include "conn.h"
#include "debug.h"
int netlink_setup(
struct proxy_dev *pdev)
{
int rvalue = 0;
/* create socket for netlink */
pdev->nl_fd = socket(PF_NETLINK, SOCK_RAW, NETDEV_PROTOCOL);
/* check if socket was actually created */
if (pdev->nl_fd < 0) {
perror("netlink_setup(socket setup)");
return -1;
}
/* allocate memory fo addreses and zero them out */
pdev->nl_src_addr = malloc(sizeof(*pdev->nl_src_addr));
pdev->nl_dst_addr = malloc(sizeof(*pdev->nl_dst_addr));
memset(pdev->nl_src_addr, 0, sizeof(*pdev->nl_src_addr));
memset(pdev->nl_dst_addr, 0, sizeof(*pdev->nl_dst_addr));
/* set up source address: this process, for bind */
pdev->nl_src_addr->nl_family = AF_NETLINK;
pdev->nl_src_addr->nl_pid = pdev->pid; /* self pid */
pdev->nl_src_addr->nl_groups = 0; /* unicast */
/* set up destination address: kernel, for later */
pdev->nl_dst_addr->nl_family = AF_NETLINK;
pdev->nl_dst_addr->nl_pid = 0; /* For Linux Kernel */
pdev->nl_dst_addr->nl_groups = 0; /* unicast */
rvalue = bind(pdev->nl_fd,
(struct sockaddr*)pdev->nl_src_addr,
sizeof(*pdev->nl_src_addr));
if (rvalue < 0) {
perror("netlink_setup(binding socket)");
return -1; /* failure */
}
printf("netlink_setup: complete\n");
/* connect is not needed since we are not using TCP but a raw socket */
return 0; /* success */
}
int netlink_send(
struct proxy_dev *pdev,
struct nlmsghdr *nlh)
{
struct msghdr msgh = {0};
struct iovec iov = {0};
if (!nlh) {
printf("netlink_send: nlh is NULL\n");
return -1; /* failure */
}
/*
debug("type: %d, pid: %d, size: %d",
nlh->nlmsg_type, nlh->nlmsg_pid, nlh->nlmsg_len);
*/
/* netlink header is our payload */
iov.iov_base = (void *)nlh;
iov.iov_len = nlh->nlmsg_len;
msgh.msg_iov = &iov; /* this normally is an array of */
msgh.msg_iovlen = 1; /* TODO, we won't always use just one */
if (sendall(pdev->nl_fd, &msgh, nlh->nlmsg_len) == -1) {
printf("netlink_send: failed to send message\n");
}
return 0; /* success */
}
int netlink_send_msg(
struct proxy_dev *pdev,
void *buff,
size_t bsize,
int msgtype,
int flags)
{
struct nlmsghdr *nlh = NULL;
nlh = malloc(NLMSG_SPACE(bsize));
if (!nlh) {
printf("netlink_send_msg: failed to allocate nlh\n");
return -1; /* failure */
}
memset(nlh, 0, NLMSG_SPACE(bsize));
nlh->nlmsg_len = NLMSG_SPACE(bsize); /* struct size + payload */
nlh->nlmsg_pid = pdev->pid;
nlh->nlmsg_type = msgtype;
/* delivery confirmation with NLMSG_ERROR and error field set to 0 */
nlh->nlmsg_flags = flags | NLM_F_ACK;
/* TODO check if we can't just assign the pointer */
if (buff) {
memcpy(NLMSG_DATA(nlh), buff, bsize);
}
if (netlink_send_nlh(pdev, nlh) == -1) {
printf("netlink_send_msg: failed to send message\n");
return -1; /* failure */
}
free(nlh);
return 0; /* success */
}
int netlink_send_nlh(
struct proxy_dev *pdev,
struct nlmsghdr *nlh)
{
struct nlmsgerr *msgerr = NULL;
int rvalue = -1;
nlh->nlmsg_pid = pdev->pid;
/* send everything */
if (netlink_send(pdev, nlh) == -1) {
printf("netlink_send_nlh: failed to send message\n");
return -1; /* failure */
}
/* get confirmation of delivery */
if ((nlh = netlink_recv(pdev)) == NULL) {
printf("netlink_send_nlh: failed to receive confirmation\n");
return -1; /* failure */
}
/* check confirmation */
if ( nlh->nlmsg_type == NLMSG_ERROR ) {
msgerr = ((struct nlmsgerr*)NLMSG_DATA(nlh));
if (msgerr->error != 0) {
debug("delivery failure, msgerr->error = %d", msgerr->error);
goto err;
}
} else {
printf("netlink_send: next message was not confirmation!\n");
goto err;
}
rvalue = 0; /* success */
err:
free(nlh);
return rvalue;
}
struct nlmsghdr * netlink_recv(
struct proxy_dev *pdev)
{
struct nlmsghdr *nlh = NULL;
struct msghdr msgh = {0};
struct iovec iov = {0};
size_t bufflen = sizeof(*nlh);
void *buffer = malloc(bufflen);
/* netlink header is our payload */
iov.iov_base = buffer;
iov.iov_len = bufflen;
msgh.msg_iov = &iov; /* this normally is an array of */
msgh.msg_iovlen = 1;
msgh.msg_flags = MSG_PEEK; /* first we need the full msg size */
/* the minimum is the size of the nlmsghdr alone */
bufflen = recvall(pdev->nl_fd, &msgh, bufflen);
if (bufflen == -1) {
printf("netlink_recv: failed to read message\n");
goto err;
}
nlh = buffer;
/*
debug("type: %d, pid: %d, size: %d",
nlh->nlmsg_type, nlh->nlmsg_pid, nlh->nlmsg_len);
*/
/* increase the buffer size if needbe */
bufflen = nlh->nlmsg_len;
buffer = realloc(buffer, bufflen);
iov.iov_base = buffer;
iov.iov_len = bufflen;
msgh.msg_flags = 0; /* get rid of MSG_PEEK */
/* get the rest of message */
bufflen = recvall(pdev->nl_fd, &msgh, bufflen);
if (bufflen == -1) {
printf("netlink_recv: failed to read message\n");
goto err;
}
nlh = buffer;
return nlh;
err:
free(buffer);
return NULL;
}
int netlink_reg_dummy_dev(
struct proxy_dev *pdev)
{
return netlink_send_msg(pdev,
pdev->dummy_dev_name,
strlen(pdev->dummy_dev_name)+1,
MSGT_CONTROL_REG_DUMMY,
NLM_F_REQUEST);
}
int netlink_reg_remote_dev(
struct proxy_dev *pdev)
{
return netlink_send_msg(pdev,
pdev->remote_dev_name,
strlen(pdev->remote_dev_name)+1,
MSGT_CONTROL_REG_SERVER,
NLM_F_REQUEST);
}
int netlink_unregister_dev(
struct proxy_dev *pdev)
{
return netlink_send_msg(pdev,
NULL, /* no payload */
0, /* 0 payload size */
MSGT_CONTROL_UNREGISTER,
NLM_F_REQUEST);
}
<file_sep>/kernel/dbg.h
#ifndef _DBG_H
#define _DBG_H
#ifdef NDEBUG
#define debug(M, ...)
#else
#define debug(M, ...) printk(KERN_DEBUG "NETDEV_DEBUG: %20s:%4d:\t\t" M "\n", __FUNCTION__, __LINE__, ##__VA_ARGS__)
#endif
#endif /* _DBG_H */
<file_sep>/include/fo_struct.h
#ifndef _FO_STRUCT_H
#define _FO_STRUCT_H
#include <linux/types.h>
#include <linux/fs.h>
/* structures used to pass file operation arguments to sending function */
struct s_fo_llseek {
loff_t offset;
int whence;
loff_t rvalue;
};
struct s_fo_read {
char __user *data;
size_t size;
loff_t offset;
ssize_t rvalue;
};
struct s_fo_write {
char __user *data;
size_t size;
loff_t offset;
ssize_t rvalue;
};
struct s_fo_aio_read {
struct kiocb *a;
const struct iovec *b;
unsigned long c;
loff_t offset;
ssize_t rvalue;
};
struct s_fo_aio_write {
struct kiocb *a;
struct iovec *b;
unsigned long c;
loff_t offset;
ssize_t rvalue;
};
struct s_dev_fo_poll {
struct poll_table_struct *wait;
unsigned long rvalue;
};
struct s_fo_unlocked_ioctl {
unsigned int cmd;
unsigned long arg;
long rvalue;
};
struct s_fo_compat_ioctl {
unsigned int cmd;
unsigned long arg;
long rvalue;
};
struct s_fo_mmap {
struct vm_area_struct *b;
int rvalue;
};
struct s_fo_open {
struct inode *inode;
int rvalue;
};
struct s_fo_flush {
int rvalue;
};
struct s_fo_release {
int rvalue;
};
struct s_fo_fsync {
loff_t b;
loff_t c;
int d;
int rvalue;
};
struct s_fo_aio_fsync {
struct kiocb *a;
int b;
int rvalue;
};
struct s_fo_fasync {
int a;
int c;
int rvalue;
};
struct s_fo_lock {
int b;
int rvalue;
};
struct s_fo_sendpage {
struct page *b;
int c;
size_t size;
loff_t *offset;
int f;
ssize_t rvalue;
};
struct s_fo_get_unmapped_area{
unsigned long b;
unsigned long c;
unsigned long d;
unsigned long e;
unsigned long rvalue;
};
struct s_fo_check_flags {
int a;
int rvalue;
};
struct s_fo_flock {
int b;
int rvalue;
};
struct s_fo_splice_write {
struct pipe_inode_info *a;
loff_t *offset;
size_t size;
unsigned int e;
ssize_t rvalue;
};
struct s_fo_splice_read {
loff_t *b;
struct pipe_inode_info *c;
size_t size;
unsigned int e;
ssize_t rvalue;
};
struct s_fo_setlease {
long b;
int rvalue;
};
struct s_fo_fallocate {
int b;
loff_t offset;
loff_t len;
long rvalue;
};
struct s_fo_show_fdinfo {
struct seq_file *a;
int rvalue;
};
#endif /* _FO_STRUCT_H */
<file_sep>/daemon/signal.h
#ifndef _SIGNAL_H
#define _SIGNAL_H
extern typedef void Sigfunc(int); /* for signal handlers */
Sigfunc * signal(int signo, Sigfunc *func);
#endif
<file_sep>/kernel/fo.c
#include <linux/module.h>
#include "fo.h"
#include "fo_send.h"
/* TODO this struct will have to be generated dynamically based on the
* operations supported by the device on the other end */
struct file_operations netdev_fops = {
.owner = THIS_MODULE,
.llseek = ndfo_send_llseek,
.read = ndfo_send_read,
.write = ndfo_send_write,
.aio_read = NULL, /* will be hard to implement because of kiocb */
.aio_write = NULL, /* will be hard to implement because of kiocb */
.readdir = NULL, /* useless for devices */
.poll = ndfo_send_poll,/* NULL assumes it's not blocing */
.unlocked_ioctl = ndfo_send_unlocked_ioctl,
.compat_ioctl = ndfo_send_compat_ioctl,
.mmap = NULL, /* for mapping device memory to user space */
.open = ndfo_send_open,
.flush = NULL, /* rarely used for devices */
.release = ndfo_send_release,
.fsync = ndfo_send_fsync,
.aio_fsync = NULL, /* will be hard to implement because of kiocb */
.fasync = ndfo_send_fasync,
.lock = NULL, /* almost never implemented */
.sendpage = NULL, /* usually not used, for sockets */
.get_unmapped_area = NULL, /* mostly NULL */
.check_flags = ndfo_send_check_flags, /* fcntl(F_SETFL...) */
.flock = ndfo_send_flock,
.splice_write = NULL, /* needs DMA */
.splice_read = NULL, /* needs DMA */
.setlease = ndfo_send_setlease,
.fallocate = ndfo_send_fallocate,
.show_fdinfo = ndfo_send_show_fdinfo
};
<file_sep>/daemon/conn.c
#include <stdio.h> /* perror */
#include <stdlib.h> /* malloc */
#include <arpa/inet.h> /* inet_pton */
#include "conn.h"
#include "protocol.h"
#include "netlink.h"
#include "debug.h"
int conn_send(struct proxy_dev *pdev, struct netdev_header *ndhead) {
struct msghdr msgh = {0};
struct iovec iov = {0};
size_t size = sizeof(*ndhead) + ndhead->size;
void *buff = NULL;
//debug("sending bytes = %zu", ndhead->size);
buff = malloc(size);
if (!buff) {
perror("conn_send(malloc)");
return -1;
}
msgh.msg_iov = &iov;
msgh.msg_iovlen = 1;
iov.iov_len = size;
iov.iov_base = buff;
memcpy(iov.iov_base, ndhead, sizeof(*ndhead));
memcpy(iov.iov_base + sizeof(*ndhead), ndhead->payload, ndhead->size);
if (sendall(pdev->rm_fd, &msgh, size) == -1) {
printf("conn_send: failed to send data\n");
free(buff);
return -1; /* failure */
}
free(buff);
return 0; /* success */
}
struct netdev_header * conn_recv(struct proxy_dev *pdev) {
struct netdev_header *ndhead = NULL;
struct msghdr msgh = {0};
struct iovec iov = {0};
int ndsize = sizeof(*ndhead);
ndhead = malloc(ndsize);
msgh.msg_iov = &iov;
msgh.msg_iovlen = 1;
iov.iov_base = (void *)ndhead;
iov.iov_len = ndsize;
if (recvall(pdev->rm_fd, &msgh, ndsize) == -1) {
printf("conn_recv: failed to read message header\n");
return NULL;
}
//debug("paylod size = %zu", ndhead->size);
ndhead->payload = malloc(ndhead->size);
iov.iov_base = ndhead->payload;
iov.iov_len = ndhead->size;
if (recvall(pdev->rm_fd, &msgh, ndhead->size) == -1) {
printf("conn_recv: failed to read rest of the message\n");
free(ndhead);
return NULL;
}
return ndhead;
}
int conn_server(struct proxy_dev *pdev) {
struct netdev_header *ndhead;
int version = NETDEV_PROTOCOL_VERSION;
int rvalue = -1;
/* create TCP/IP socket for connection with server */
pdev->rm_fd = socket(AF_INET, SOCK_STREAM, 0);
if (pdev->rm_fd == -1) {
perror("conn_server(socket)");
return -1;
}
pdev->rm_addr = malloc(sizeof(*pdev->rm_addr));
if (!pdev->rm_addr) {
perror("conn_server(malloc)");
return -1;
}
pdev->rm_addr->sin_family = AF_INET;
pdev->rm_addr->sin_port = htons(pdev->rm_portaddr);
inet_pton(AF_INET, (void*)pdev->rm_ipaddr, &pdev->rm_addr->sin_addr);
printf("conn_server: connecting to: %s:%d\n",
pdev->rm_ipaddr, pdev->rm_portaddr);
rvalue = connect(pdev->rm_fd,
(struct sockaddr*)pdev->rm_addr,
sizeof(*pdev->rm_addr));
if (rvalue == -1) {
perror("conn_server(connect)");
return -1;
}
ndhead = malloc(sizeof(*ndhead));
if (!ndhead) {
perror("conn_server(malloc)");
return -1;
}
ndhead->msgtype = MSGT_CONTROL_VERSION;
ndhead->size = sizeof(version);
ndhead->payload = malloc(ndhead->size);
memcpy(ndhead->payload, &version, ndhead->size);
/* send version */
if (conn_send(pdev, ndhead) == -1) {
printf("conn_server: failed to send version\n");
goto err;
}
free(ndhead->payload);
free(ndhead);
/* receive reply */
ndhead = conn_recv(pdev);
if (!ndhead) {
printf("conn_server: failed to receive version reply\n");
goto err;
}
if (ndhead->size != sizeof(int)) {
printf("conn_server: wrong payload size\n");
goto err;
}
version = *((int*)ndhead->payload);
if (version != 0) {
printf("conn_server: wrong version = %d\n", version);
goto err;
}
printf("conn_server: connection successful, version correct = %d\n",
version);
rvalue = 0; /* success */
err:
free(ndhead->payload);
free(ndhead);
return rvalue;
}
int conn_client(struct proxy_dev *pdev) {
struct netdev_header *ndhead;
int rvalue = -1;
int version;
int reply = NETDEV_PROTOCOL_SUCCESS; /* success = 0 */
ndhead = conn_recv(pdev);
if (ndhead->msgtype != MSGT_CONTROL_VERSION) {
printf("conn_client: wrong message type!\n");
}
if (ndhead->size != sizeof(int)) {
printf("conn_client: wrong payload size!\n");
return -1; /* failure */
}
version = *((int*)ndhead->payload);
printf("conn_client: received version = %d\n", version);
/* check version number */
if (version != NETDEV_PROTOCOL_VERSION) {
printf("conn_client: wrong protocol version = %d\n", version);
reply = NETDEV_PROTOCOL_VERSION;
}
memcpy(ndhead->payload, &reply, sizeof(int));
if (conn_send(pdev, ndhead) == -1) {
printf("conn_client: failed to send version reply\n");
goto err;
}
printf("conn_client: protocol version correct = %d\n", version);
rvalue = reply;
err:
free(ndhead->payload);
free(ndhead);
return rvalue; /* success */
}
int conn_send_dev_reg(struct proxy_dev *pdev) {
struct netdev_header *ndhead;
int rvalue = 0;
ndhead = malloc(sizeof(*ndhead));
if (!ndhead) {
perror("conn_send_dev_reg(malloc)");
return -1;
}
ndhead->msgtype = MSGT_CONTROL_REG_SERVER;
ndhead->size = strlen(pdev->remote_dev_name)+1; /* plus null */
ndhead->payload = pdev->remote_dev_name;
printf("conn_send_dev_reg: sending device name: %s\n",
pdev->remote_dev_name);
if (conn_send(pdev, ndhead) == -1) {
printf("conn_send_dev_reg: failed to send device registration\n");
free(ndhead);
return -1;
}
free(ndhead);
ndhead = conn_recv(pdev);
if (!ndhead) {
printf("conn_send_dev_reg: failed to receive reply\n");
return -1;
}
rvalue = *((int*)ndhead->payload);
if (rvalue != NETDEV_PROTOCOL_SUCCESS) {
printf("conn_send_dev_reg: server failed to register device\n");
return -1;
}
if (netlink_reg_dummy_dev(pdev) == -1) {
printf("proxy_client: failed to register device on the server!\n");
return -1;
}
printf("conn_send_dev_reg: device registered\n");
return 0; /* success */
}
int conn_recv_dev_reg(struct proxy_dev *pdev) {
struct netdev_header *ndhead;
int reply = NETDEV_PROTOCOL_SUCCESS;
ndhead = conn_recv(pdev);
if (ndhead->msgtype != MSGT_CONTROL_REG_SERVER) {
printf("conn_recv_dev_reg: wrong message type!\n");
return -1; /* failure */
}
pdev->remote_dev_name = malloc(ndhead->size);
if (!pdev->remote_dev_name) {
perror("conn_recv_dev_reg(malloc)");
return -1;
}
memcpy(pdev->remote_dev_name, ndhead->payload, ndhead->size);
debug("registering device: %s", pdev->remote_dev_name);
if (netlink_reg_remote_dev(pdev) == -1) {
printf("conn_recv_dev_reg: failed to register device: %s\n",
pdev->remote_dev_name);
return -1;
}
ndhead->payload = malloc(sizeof(reply));
memcpy(ndhead->payload, &reply, sizeof(reply));
if (conn_send(pdev, ndhead) == -1) {
printf("conn_recv_dev_reg: failed to send reply\n");
return -1;
}
free(ndhead->payload);
return 0; /* success */
}
/* size is the minimum that should be read */
int recvall(int conn_fd, struct msghdr *msgh, int size) {
int rvalue = 0, bytes = 0;
do {
rvalue = recvmsg(conn_fd, msgh, msgh->msg_flags);
//debug("rvalue = %d", rvalue);
if (rvalue == -1) {
perror("recvall(recvmsg)");
return -1; /* falure */
} else if (rvalue == 0 ) {
return -1;
}
bytes += rvalue;
msgh->msg_iov->iov_base += rvalue;
msgh->msg_iov->iov_len = bytes;
} while (bytes < size);
return bytes; /* success */
}
int sendall(int conn_fd, struct msghdr *msgh, int size) {
int rvalue = 0, bytes = 0;
do {
rvalue = sendmsg(conn_fd, msgh, msgh->msg_flags);
//debug("rvalue = %d", rvalue);
if (rvalue == -1) {
perror("sendmsg(sendmsg)");
return -1; /* falure */
} else if (rvalue == 0 ) {
return -1;
}
bytes += rvalue;
msgh->msg_iov->iov_base += rvalue;
msgh->msg_iov->iov_len = bytes;
} while (bytes < size);
return 0; /* success */
}
<file_sep>/daemon/conn.h
#ifndef _CONN_H
#define _CONN_H
#include "proxy.h"
#include "netprotocol.h"
int conn_send(struct proxy_dev *pdev, struct netdev_header *ndhead);
struct netdev_header * conn_recv(struct proxy_dev *pdev);
int conn_server(struct proxy_dev *pdev);
int conn_client(struct proxy_dev *pdev);
int conn_send_dev_reg(struct proxy_dev *pdev);
int conn_recv_dev_reg(struct proxy_dev *pdev);
int recvall(int conn_fd, struct msghdr *msgh, int size);
int sendall(int conn_fd, struct msghdr *msgh, int size);
#endif
<file_sep>/daemon/main.c
#include <string.h> /* memset, memcopy, memmove, memcmp */
#include <stdio.h> /* printf */
#include <stdlib.h> /* malloc */
#include <unistd.h> /* close, getpid */
#include <sys/socket.h> /* socklen_t */
#include <sys/types.h> /* pid_t */
#include <sys/wait.h> /* waitpid */
#include <arpa/inet.h> /* inet_notp */
#include <errno.h>
#include <netinet/ip.h>
#include <signal.h> /* signal */
#include "signal.h"
#include "proxy.h"
#include "protocol.h"
#include "debug.h"
void parent_sig_chld(int signo) {
pid_t pid;
int stat;
printf("sig_chld: received signal\n");
while ( (pid = waitpid(-1, &stat, WNOHANG)) > 0 ) {
/* TODO should't use printf in signal handling */
printf("ALERT: child %d terminated\n", pid);
}
return;
}
int netdev_listener(
int port)
{
int listenfd, connfd;
int rvalue;
pid_t childpid;
socklen_t clilen;
struct sockaddr_in cliaddr, servaddr;
char str[INET_ADDRSTRLEN];
void *ptr;
listenfd = socket(AF_INET, SOCK_STREAM, 0);
if ( listenfd < 0 ) {
perror("netdev_listener: failed to create socket");
return -1;
}
memset(&servaddr, 0, sizeof(servaddr));
servaddr.sin_family = AF_INET;
servaddr.sin_addr.s_addr = htonl(INADDR_ANY);
servaddr.sin_port = htons(port);
rvalue = bind(listenfd,
(struct sockaddr*)&servaddr,
sizeof(servaddr));
if ( rvalue < 0 ) {
perror("netdev_listener: failed to bind to address");
return -1;
}
rvalue = listen(listenfd, NETDEV_LISTENQ);
if ( rvalue < 0 ) {
perror("netdev_listener: failed to listen");
return -1;
}
clilen = sizeof(cliaddr);
printf("netdev_listener: starting listener at port: %d\n", port);
while (1) {
connfd = accept(listenfd, (struct sockaddr *) &cliaddr, &clilen);
if (connfd < 0) {
if (errno == EINTR) {
continue;
} else {
perror("netdev_listener: accept error");
}
}
ptr = (char *)inet_ntop(cliaddr.sin_family ,
&cliaddr.sin_addr,
str,
sizeof(str));
if ( ptr != NULL ) {
printf("netdev_listener: new connection from %s:%d\n",
str,
ntohs(cliaddr.sin_port));
}
/* fork for every new client and serve it */
if ( (childpid = fork()) ==0 ) {
/* decrease the counter for listening socket,
* this process won't use it */
close(listenfd);
proxy_server(connfd);
exit(0);
}
/* decreas the counter for new connection */
close(connfd);
}
return 0;
}
struct proxy_dev ** read_config(char *filename, int *count) {
struct proxy_dev **pdevs = NULL;
FILE *fp;
char c;
char line[100];
int i = 0;
int rvalue;
*count = 0;
if (filename == NULL) {
return NULL;
}
fp = fopen(filename, "r");
if (!fp) {
perror("failed to open file");
return NULL;
}
while (EOF != (fscanf(fp, "%c%*[^\n]", &c), fscanf(fp, "%*c"))) {
if (c != '#') { /* ignore comments */
++*count;
}
}
rewind(fp); /* go back to the beginning of the file */
pdevs = malloc(*count * sizeof(struct proxy_dev*));
memset(pdevs, 0, *count * sizeof(struct proxy_dev*));
if (!pdevs) {
perror("read_config: failed to allocate pdevs");
return NULL; /* failure */
}
for (i = 0; i < *count; ) {
fgets(line, sizeof(line), fp);
if (line[0] == '#') { /* if first character is # it's a comment */
continue;
}
if (!pdevs[i]) {
pdevs[i] = malloc(sizeof(struct proxy_dev));
memset(pdevs[i], 0, sizeof(struct proxy_dev));
pdevs[i]->remote_dev_name = malloc(NETDEV_MAX_STRING);
pdevs[i]->dummy_dev_name = malloc(NETDEV_MAX_STRING);
pdevs[i]->rm_ipaddr = malloc(NETDEV_MAX_STRING);
}
pdevs[i]->client = true; /* this is a client instance */
rvalue = sscanf(line, "%20[^;];%20[^;];%20[^;];%d\n",
pdevs[i]->remote_dev_name,
pdevs[i]->dummy_dev_name,
pdevs[i]->rm_ipaddr,
&pdevs[i]->rm_portaddr);
if (rvalue == EOF) {
break;
}
if (rvalue < 4) {
printf("failed to parse configuration in line %d\n", i+1);
break;
}
i++;
}
*count = i;
return pdevs; /* success */
}
int main(int argc, char *argv[]) {
int pid, dev_count, i, port = NETDEV_DAEMON_PORT;
char *conffile = NULL;
char c;
struct proxy_dev **pdevs = NULL;
while ((c = getopt(argc, argv, ":hf:p:")) != -1) {
switch(c) {
case 'p':
port = atoi(optarg);
break;
case 'f':
conffile = optarg;
break;
case 'h':
printf("usage: %s [-f CONFIGFILE] [-p SERVERPORT] [-h]\n", argv[0]);
return 0;
case ':': /* -f or -o without operand */
fprintf(stderr,
"Option -%c requires an operand\n", optopt);
return 1;
case '?':
fprintf(stderr,
"Unrecognized option: '-%c'\n", optopt);
return 1;
}
}
/* to run waitpid for all forked proxies */
signal(SIGCHLD, parent_sig_chld);
if (!(pdevs = read_config(conffile, &dev_count))) {
printf("failed to client read configuration\n");
} else {
for ( i = 0 ; i < dev_count ; i++ ) {
if ( ( pid = fork() ) == 0 ) {
proxy_client(pdevs[i]);
exit(0);
} else if ( pid < 0 ) {
perror("main: failed to fork");
}
}
}
if ( netdev_listener(port) ) {
printf("main: could not start listener\n");
}
return 0;
}
<file_sep>/kernel/netlink.h
#ifndef _NETLINK_H
#define _NETLINK_H
#include <linux/skbuff.h>
#include "netdevmgm.h"
#include "fo.h"
void prnttime(void);
int netlink_echo(int pid, int seq, char *msg);
void netlink_recv(struct sk_buff *skb);
int netlink_create_dummy(int pid, int seq, char *buff, size_t bsize);
int netlink_ackmsg(struct netdev_data *nddata, int seq, short msgtype);
int netlink_send(struct netdev_data *nddata, int seq, short msgtype, int flags, void *buff, size_t bufflen);
int netlink_send_msg( int nlpid, struct nlmsghdr *nlh, int flags, void *buff, size_t bufflen);
int netlink_send_skb(struct netdev_data *nddata, struct sk_buff *skb);
struct sk_buff * netlink_pack_skb( struct nlmsghdr *nlh, void *buff, size_t bufflen);
int netlink_send_fo(struct netdev_data *nddata, struct fo_req *req);
int netlink_init(void);
void netlink_exit(void);
#endif /* _NETLINK_H */
<file_sep>/kernel/main.c
#include <linux/compiler.h>
#include <linux/module.h> /* for MODULE_ macros */
#include <linux/version.h>
#include <linux/kernel.h>
#include <linux/types.h>
#include <linux/kdev_t.h>
#include <linux/fs.h>
#include "protocol.h"
#include "netlink.h"
#include "netdevmgm.h"
#include "fo.h"
MODULE_VERSION("0.0.1");
MODULE_LICENSE("GPL v2");
MODULE_AUTHOR("<NAME> <panswiata_at_<EMAIL>_com>");
MODULE_DESCRIPTION("This is an experimental kernel driver. Please use at your own risk.");
static int dev_count = NETDEV_MAX_DEVICES; /* TODO driver option */
static int netdev_major = NETDEV_MAJOR; /* TODO driver option */
dev_t netdev_devno;
struct class *netdev_class;
static void netdev_cleanup(void)
{
while (ndmgm_end()) {
printk(KERN_ERR "netdev_cleanup: failed to delete devices");
}
if (netdev_class)
class_destroy(netdev_class);
/* netdev_cleanup is not called if alloc_chrdev_region has failed */
/* free the major and minor numbers */
unregister_chrdev_region(netdev_devno, dev_count);
printk(KERN_DEBUG "netdev: driver cleanup finished\n");
return;
}
static int __init netdev_init(void)
{
int err;
int first_minor = 0;
/* get a range of minor numbers (starting with 0) to work with
* we are using alloc rather than register to get dynamic major */
err = alloc_chrdev_region(&netdev_devno, first_minor, dev_count, NETDEV_NAME);
/* fail gracefully if need be */
if (err) {
printk(KERN_ERR "netdev: error registering chrdev!\n");
return err;
}
netdev_major = MAJOR(netdev_devno);
netdev_class = class_create(THIS_MODULE, NETDEV_NAME);
if (IS_ERR(netdev_class)) {
err = PTR_ERR(netdev_class);
goto fail;
}
/* create array and hashtable for devices */
ndmgm_prepare();
/* setup netlink */
err = netlink_init();
if (err) {
printk(KERN_ERR "netdev: netlink setup failed!\n");
goto fail;
}
return 0; /* success */
fail:
netdev_cleanup();
return err;
}
static void __exit netdev_exit(void)
{
netlink_exit();
netdev_cleanup();
return;
}
module_exit(netdev_exit);
module_init(netdev_init);
<file_sep>/kernel/fo_send.h
#ifndef _FO_SEND_H
#define _FO_SEND_H
#include <linux/fs.h> /* fl_owner_t */
/* functions for sending and receiving file operations */
loff_t ndfo_send_llseek(struct file *flip, loff_t offset, int whence);
ssize_t ndfo_send_read(struct file *flip, char __user *data, size_t size, loff_t *offset);
ssize_t ndfo_send_write(struct file *flip, const char __user *data, size_t size, loff_t *offset);
size_t ndfo_send_aio_read(struct kiocb *a, const struct iovec *b, unsigned long c, loff_t offset);
ssize_t ndfo_send_aio_write(struct kiocb *a, const struct iovec *b, unsigned long c, loff_t d);
unsigned int ndfo_send_poll(struct file *filp, struct poll_table_struct *wait);
long ndfo_send_unlocked_ioctl(struct file *filp, unsigned int b, unsigned long c);
long ndfo_send_compat_ioctl(struct file *filp, unsigned int b, unsigned long c);
int ndfo_send_mmap(struct file *filp, struct vm_area_struct *b);
int ndfo_send_open(struct inode *inode, struct file *filp);
int ndfo_send_flush(struct file *filp, fl_owner_t id);
int ndfo_send_release(struct inode *a, struct file *filp);
int ndfo_send_fsync(struct file *filp, loff_t b, loff_t offset, int d);
int ndfo_send_aio_fsync(struct kiocb *a, int b);
int ndfo_send_fasync(int a, struct file *filp, int c);
int ndfo_send_lock(struct file *filp, int b, struct file_lock *c);
ssize_t ndfo_send_sendpage(struct file *filp, struct page *b, int c, size_t size, loff_t *offset, int f);
unsigned long ndfo_send_get_unmapped_area(struct file *filp, unsigned long b, unsigned long c,unsigned long d, unsigned long e);
int ndfo_send_check_flags(int a);
int ndfo_send_flock(struct file *filp, int b, struct file_lock *c);
ssize_t ndfo_send_splice_write(struct pipe_inode_info *a, struct file *filp, loff_t *offset, size_t size, unsigned int e);
ssize_t ndfo_send_splice_read(struct file *filp, loff_t *offset, struct pipe_inode_info *c, size_t size, unsigned int e);
int ndfo_send_setlease(struct file *filp, long b, struct file_lock **c);
long ndfo_send_fallocate(struct file *filp, int b, loff_t offset, loff_t len);
int ndfo_send_show_fdinfo(struct seq_file *a, struct file *filp);
#endif /* _FO_SEND_H */
<file_sep>/include/protocol.h
#ifndef _PROTOCOL_H
#define _PROTOCOL_H
/* this number HAS TO BE incremented every time anything in the
* the core protocol is changed */
#define NETDEV_PROTOCOL_VERSION 2
#define NETDEV_PROTOCOL_SUCCESS 0 /* 0 will always mean correct */
#define NETDEV_PROTOCOL_FAILURE -1 /* -1 will always mean failure */
/* size limit of messages sent through sendmsg */
#define NETDEV_MESSAGE_LIMIT 213000
/* defines the default daemon port */
#define NETDEV_DAEMON_PORT 9999
/* 2nd argument to listen(), max size of listen queue */
#define NETDEV_LISTENQ 1024
/* defines the netlink bus used, we want our own protocol so we use the
* last one of the 32 available buses */
#define NETDEV_PROTOCOL 31
/* define type of message for netlink driver control,
* will be used in nlmsghdr->nlmsg_type */
#define MSGT_CONTROL_ECHO 1 /* test to check if diver works */
#define MSGT_CONTROL_SUCCESS 2 /* test to check if diver works */
#define MSGT_CONTROL_FAILURE 3 /* test to check if diver works */
#define MSGT_CONTROL_VERSION 4 /* check if driver is compatible */
#define MSGT_CONTROL_REG_DUMMY 5 /* register a new device */
#define MSGT_CONTROL_REG_SERVER 6 /* register a new device */
#define MSGT_CONTROL_UNREGISTER 7 /* unregister the device */
#define MSGT_CONTROL_RECOVER 8 /* recover a device after lost conn. */
#define MSGT_CONTROL_DRIVER_STOP 9 /* close all devices and stop driver */
#define MSGT_CONTROL_LOSTCONN 10 /* lost connection with other end */
/* limit of control messsages */
#define MSGT_CONTROL_START 0
#define MSGT_CONTROL_END 11
/* Definitions used to distinguis between file operation structures
* that contain all the functiona arguments, best used with short or __u16
* Will be either used in nlmsghdr->nlmsg_type or some inner variable */
#define MSGT_FO_LLSEEK 101
#define MSGT_FO_READ 102
#define MSGT_FO_WRITE 103
#define MSGT_FO_AIO_READ 104
#define MSGT_FO_AIO_WRITE 105
#define MSGT_FO_READDIR 106
#define MSGT_FO_POLL 107
#define MSGT_FO_UNLOCKED_IOCTL 108
#define MSGT_FO_COMPAT_IOCTL 109
#define MSGT_FO_MMAP 110
#define MSGT_FO_OPEN 111
#define MSGT_FO_FLUSH 112
#define MSGT_FO_RELEASE 113
#define MSGT_FO_FSYNC 114
#define MSGT_FO_AIO_FSYNC 115
#define MSGT_FO_FASYNC 116
#define MSGT_FO_LOCK 117
#define MSGT_FO_SENDPAGE 118
#define MSGT_FO_GET_UNMAPPED_AREA 119
#define MSGT_FO_CHECK_FLAGS 120
#define MSGT_FO_FLOCK 121
#define MSGT_FO_SPLICE_WRITE 122
#define MSGT_FO_SPLICE_READ 123
#define MSGT_FO_SETLEASE 124
#define MSGT_FO_FALLOCATE 125
#define MSGT_FO_SHOW_FDINF 126
#define MSGT_FO_START 100
#define MSGT_FO_END 127
#endif /* _PROTOCOL_H */
<file_sep>/daemon/proxy.h
#ifndef _PROXY_H
#define _PROXY_H
#include <linux/netlink.h> /* sockaddr_nl */
#include <netinet/in.h> /* sockaddr_in */
#include <sys/un.h> /* sockaddr_un */
#define NETDEV_MAX_STRING 20
typedef enum {
true,
false
} bool;
struct proxy_dev {
int pid; /* pid od the proxy process */
int nl_fd; /* netlink file descryptor */
int rm_fd; /* remote file descryptor */
int us_fd[2]; /* unix socket pair, first one is for reading */
bool client; /* defines if this is a client or a server */
struct sockaddr_nl *nl_src_addr;
struct sockaddr_nl *nl_dst_addr;
struct sockaddr_in *rm_addr;
struct sockaddr_un *us_addr;
int rm_portaddr;
char *rm_ipaddr;
char *remote_dev_name;
char *dummy_dev_name;
};
int proxy_netlink_setup(struct proxy_dev *pdev);
int proxy_register_device(struct proxy_dev *pdev, struct msghdr *p_msg, struct iovec *p_iov);
int proxy_unregister_device(void);
void proxy_setup_signals();
int proxy_setup_unixsoc(struct proxy_dev *pdev);
struct proxy_dev * proxy_alloc_dev(int connfd);
void proxy_client(struct proxy_dev *pdev);
void proxy_server(int connfd);
void proxy_sig_hup(int signo);
int proxy_loop();
int proxy_control(struct proxy_dev *pdev);
int proxy_handle_remote(struct proxy_dev *pdev);
int proxy_handle_netlink(struct proxy_dev *pdev);
int netlink_unregister_dev(struct proxy_dev *pdev);
#endif
<file_sep>/kernel/netdevmgm.h
#ifndef _NETDEVMGM_H
#define _NETDEVMGM_H
#include <linux/cdev.h>
#include <linux/module.h> /* for THIS_MODULE */
#include <linux/hashtable.h>
#include <linux/rwsem.h>
#include <linux/kfifo.h>
#include <linux/slab.h>
#include "netdev.h"
#include "protocol.h"
#include "fo_comm.h"
/* necessary since netdevmgm.h and fo_comm.h reference each other */
struct fo_req;
/* __u32, unsigned 32bit type, 12 bit for majro, 20 minor */
extern dev_t netdev_devno;
extern struct class *netdev_class;
struct netdev_data {
unsigned int nlpid; /* pid of the process that created this device */
bool active; /* set to false if netlink connection is lost */
bool dummy; /* defines if this is a server device or a dummy one */
char *devname; /* name of the device provided by the process */
atomic_t users; /* counter of threads using this objecto */
atomic_t curseq; /* last used sequence number for this device */
spinlock_t nllock; /* netlink lock ensuring proper order for ACK msg */
struct cdev *cdev; /* internat struct representing char devices */
struct device *device;
struct hlist_node hnode; /* to use with hastable */
struct rw_semaphore sem; /* necessary since threads can use this */
struct kmem_cache *queue_pool; /* pool of memory for fo_req structs */
/* hash table for active open files */
DECLARE_HASHTABLE(foacc_htable, NETDEV_HTABLE_ACC_SIZE);
};
struct netdev_data * ndmgm_alloc_data(int nlpid, char *name, int minor);
int ndmgm_create_dummy(int nlpid, char *name);
int ndmgm_create_server(int nlpid, char *name);
void ndmgm_free_data(struct netdev_data *nddata);
struct netdev_data * ndmgm_find(int nlpid);
void ndmgm_put_minor(int minor);
int ndmgm_get_minor(int pid);
int ndmgm_find_pid(dev_t dev);
int ndmgm_incseq(struct netdev_data *nddata);
struct fo_access * ndmgm_find_acc(struct netdev_data *nddata, int access_id);
int ndmgm_find_destroy(int nlpid);
int ndmgm_destroy(struct netdev_data *nddata);
int ndmgm_destroy_allacc( struct netdev_data *nddata);
int ndmgm_destroy_dummy(struct netdev_data *nddata);
int ndmgm_destroy_server(struct netdev_data *nddata);
int ndmgm_end(void);
void ndmgm_prepare(void);
void ndmgm_get(struct netdev_data *nddata);
void ndmgm_put(struct netdev_data *nddata);
int ndmgm_refs(struct netdev_data *nddata);
#endif
<file_sep>/daemon/signal.c
#include <sys/types.h> /* pid_t */
#include <sys/wait.h> /* waitpid */
#include <stdio.h> /* TODO should use write when handling signal */
#include <signal.h>
#include <unistd.h>
#include "signal.h"
typedef void Sigfunc(int); /* for signal handlers */
Sigfunc * signal(int signo, Sigfunc *func) {
struct sigaction act, oact;
act.sa_handler = func;
sigemptyset(&act.sa_mask);
act.sa_flags = 0;
if (signo == SIGALRM) {
act.sa_flags |= SA_RESTART;
}
if (sigaction(signo, &act, &oact) < 0)
return SIG_ERR;
return oact.sa_handler;
}
<file_sep>/daemon/Makefile
CC = gcc
CFLAGS = -g -Wall
INCLUDE = -I../include -I./lib
DEPS = ../include/protocol.h
OBJ = netlink.o conn.o signal.o proxy.o main.o
TARGET = netdevd
all: $(TARGET)
%.o: %.c $(DEPS)
$(CC) $(CFLAGS) $(INCLUDE) -c -o $@ $<
$(TARGET): $(OBJ)
$(CC) $(CFLAGS) -o $(TARGET) $^
clean:
rm ./${TARGET} ./*.o
<file_sep>/README.md
netdev
======
Description
-----------
This project has a goal of allowing the sharing of physical devices like character and block devices between linux machines over TCP/IP.
Disclamer
---------
The code is not yet stable. Test at your own peril. This WILL cause kernel panics.
Usage
-----
Both kernel module and the daemon program can be compiled with make.
Daemon can be started with or without arguments.
Usage: ./netdevd [-f CONFIGFILE] [-p LISTENPORT] [-h]
Format of configuration file:
RemoteDevicePath;DummyDeviceName;DaemonIP;DaemonPort
Example:
/dev/urandom;netdev_urandom;192.168.1.13;9999
Some static limitations apply. For example limit of devices is 32 and is defined in include/protocol.h with NETDEV_MAX_DEVICES and NETDEV_HTABLE_DEV_SIZE.
Notes
-----
If you want to play with this software please do, and please report any crashes and bugs you might encounter to my github at https://github.com/PonderingGrower/netdev .
<file_sep>/kernel/Makefile
# Makefile – makefile of our first driver
TARGET := netdev
DEBUG := -g
WARN := -Wall -Wstrict-prototypes -Wmissing-prototypes
NOWARN := -Wno-unused-function
INCLUDE := -I$(src)/../include/
ccflags-y := -O ${WARN} ${NOWARN} ${DEBUG} ${INCLUDE}
# if KERNELRELEASE is defined, we've been invoked from the
# kernel build system and can use its language.
ifneq (${KERNELRELEASE},)
obj-m := ${TARGET}.o
${TARGET}-objs := fo_access.o fo_send.o fo_recv.o fo_comm.o fo.o netdevmgm.o netlink.o main.o
# Otherwise we were called directly from the command line.
# Invoke the kernel build system.
else
KERNEL_SOURCE := /usr/src/linux
PWD := $(shell pwd)
default:
${MAKE} -C ${KERNEL_SOURCE} M=${PWD} modules
clean:
${MAKE} -C ${KERNEL_SOURCE} M=${PWD} clean
install:
${MAKE} -C ${KERNEL_SOURCE} M=${PWD} modules_install
endif
<file_sep>/daemon/netlink.h
#ifndef _NETLINK_H
#define _NETLINK_H
#include "proxy.h"
int netlink_setup(struct proxy_dev *pdev);
int netlink_reg_dummy_dev(struct proxy_dev *pdev);
int netlink_reg_remote_dev(struct proxy_dev *pdev);
int netlink_send(struct proxy_dev *pdev, struct nlmsghdr *nlh);
int netlink_send_msg(struct proxy_dev *pdev, void *buff, size_t bsize, int msgtype, int flags);
int netlink_send_nlh(struct proxy_dev *pdev, struct nlmsghdr *nlh);
struct nlmsghdr * netlink_recv(struct proxy_dev *pdev);
#endif
<file_sep>/kernel/netlink.c
#include <linux/netlink.h> /* for netlink sockets */
#include <linux/module.h>
#include <net/sock.h>
#include <linux/kthread.h> /* task_struct, kthread_run */
#include <linux/ktime.h> /* getnstimeofday */
#include "netlink.h"
#include "netdevmgm.h"
#include "protocol.h"
#include "dbg.h"
struct sock *nl_sk = NULL;
void prnttime(void) {
struct timespec ts;
getnstimeofday(&ts);
printk(KERN_DEBUG " -- %pS - TIME: sec %ld, nsec %ld\n",
__builtin_return_address(0),
ts.tv_sec, ts.tv_nsec);
}
void netlink_recv(struct sk_buff *skb)
{
struct netdev_data *nddata = NULL;
struct task_struct *task = NULL;
struct nlmsghdr *nlh = NULL;
int pid, msgtype, err = 0;
void *data;
nlh = nlmsg_hdr(skb);
pid = nlh->nlmsg_pid;
msgtype = nlh->nlmsg_type;
//debug("type: %d, pid: %d, size: %d", msgtype, pid, nlh->nlmsg_len);
if ( pid == 0 ) {
printk(KERN_ERR "netlink_recv: message from kernel");
return;
}
if (msgtype >= MSGT_CONTROL_START && msgtype < MSGT_CONTROL_END) {
switch (msgtype) {
case MSGT_CONTROL_ECHO:
err = netlink_send_msg(pid, nlh, NLM_F_ECHO,
NLMSG_DATA(nlh),
sizeof(int));
break;
case MSGT_CONTROL_VERSION:
msgtype = NETDEV_PROTOCOL_VERSION,
err = netlink_send_msg(pid, nlh, NLM_F_ECHO,
&msgtype,
sizeof(msgtype));
break;
case MSGT_CONTROL_REG_DUMMY:
err = ndmgm_create_dummy(pid, (char*)nlmsg_data(nlh));
break;
case MSGT_CONTROL_REG_SERVER:
err = ndmgm_create_server(pid, (char*)nlmsg_data(nlh));
break;
case MSGT_CONTROL_UNREGISTER:
err = ndmgm_find_destroy(pid);
break;
default:
printk(KERN_ERR
"netlink_recv: unknown control msg type: %d\n",
msgtype);
break;
}
} else if ( msgtype > MSGT_FO_START && msgtype < MSGT_FO_END ) {
/* if it's file operation device must exist */
nddata = ndmgm_find(pid);
if (!nddata) {
printk(KERN_ERR "netlink_recv: failed to find nddata\n");
return;
}
/* we will use this skb for ACK*/
data = skb_copy(skb, GFP_KERNEL);
/* create a new thread so server doesn't wait for ACK */
task = kthread_create(&fo_recv, data, "fo_recv");
if (IS_ERR(task)) {
printk(KERN_ERR
"fo_recv: failed to create thread, error = %ld\n",
PTR_ERR(task));
err = PTR_ERR(task); /* failure */
}
/* when lock is before kthread_run it creates "spinlock wrong CPU"
* error on the first open operation, that's why kthread_creat
* and wake_up_pprocess is used */
spin_lock(&nddata->nllock); /* make sure we send ACK first */
/* start the thread */
wake_up_process(task);
} else {
debug("unknown message type: %d", msgtype);
}
/* if error or if this message says it wants a response */
if ( err || (nlh->nlmsg_flags & NLM_F_ACK )) {
/* send messsage,nlmsg_unicast will take care of freeing skb */
netlink_ack(skb, nlh, err); /* err should be 0 when no error */
} else {
dev_kfree_skb(skb);
}
/* if nddata is NULL the spinlock is not locked */
if (nddata) {
spin_unlock(&nddata->nllock);
}
}
int netlink_send(
struct netdev_data *nddata,
int seq,
short msgtype,
int flags,
void *buff,
size_t bufflen)
{
struct nlmsghdr *nlh;
struct sk_buff *skb;
int rvalue;
//debug("type: %d, pid: %d, size: %zu", msgtype, nddata->nlpid, bufflen);
if (!nddata) {
printk(KERN_ERR "netlink_send: nddata is NULL\n");
return -1;
}
/* allocate space for message header and it's payload */
skb = nlmsg_new(bufflen, GFP_KERNEL);
if(!skb) {
printk(KERN_ERR "netlink_send: failed to allocate new sk_buff!\n");
goto free_skb;
}
/* set pid, seq, message type, payload size and flags */
nlh = nlmsg_put(
skb,
nddata->nlpid,
seq,
msgtype,
bufflen, /* adds nlh size (16bytes) for full size */
flags | NLM_F_ACK); /* for delivery confirmation */
if (!nlh) {
printk(KERN_ERR "netlink_send: failed to create nlh\n");
goto free_skb;
}
NETLINK_CB(skb).portid = nddata->nlpid;
NETLINK_CB(skb).dst_group = 0; /* not in mcast group */
if (buff) { /* possible to send message without payload */
memcpy(nlmsg_data(nlh) ,buff ,bufflen);
}
spin_lock(&nddata->nllock); /* to make sure ACK is sent first */
/* send messsage,nlmsg_unicast will take care of freeing skb */
rvalue = nlmsg_unicast(nl_sk, skb, nddata->nlpid);
spin_unlock(&nddata->nllock);
/* TODO get confirmation of delivery */
if(rvalue < 0) {
printk(KERN_INFO "netlink_send: error while sending back to user\n");
return -1; /* failure */
}
return 0; /* success */
free_skb:
nlmsg_free(skb);
return -1;
}
int netlink_send_msg(
int nlpid,
struct nlmsghdr *nlh,
int flags,
void *buff,
size_t bufflen)
{
return netlink_send(ndmgm_find(nlpid),
nlh->nlmsg_seq,
nlh->nlmsg_type,
flags,
buff,
bufflen);
}
int netlink_send_skb(
struct netdev_data *nddata,
struct sk_buff *skb)
{
int rvalue;
if (!skb) {
printk(KERN_ERR "netlink_send: skb is null\n");
return -1;
}
/*
debug("type: %d, pid: %d, size: %d",
nlmsg_hdr(skb)->nlmsg_type,
nddata->nlpid,
nlmsg_hdr(skb)->nlmsg_len);
*/
NETLINK_CB(skb).portid = nddata->nlpid;
spin_lock(&nddata->nllock); /* to make sure ACK is sent first */
/* send messsage,nlmsg_unicast will take care of freeing skb */
rvalue = nlmsg_unicast(nl_sk, skb, nddata->nlpid);
/* send messsage,nlmsg_unicast will take care of freeing skb */
spin_unlock(&nddata->nllock);
if(rvalue < 0) {
printk(KERN_INFO "netlink_send: error while sending back to user\n");
return -1; /* failure */
}
return 0; /* success */
}
struct sk_buff * netlink_pack_skb(
struct nlmsghdr *nlh,
void *buff,
size_t bufflen)
{
struct sk_buff *skb;
nlh->nlmsg_len = NLMSG_SPACE(bufflen);
skb = alloc_skb(nlh->nlmsg_len, GFP_KERNEL);
if (!skb) {
printk(KERN_ERR "fo_exectue: failed to expand skb\n");
return NULL;
}
nlh = nlmsg_put(
skb,
nlh->nlmsg_pid,
nlh->nlmsg_seq,
nlh->nlmsg_type,
bufflen,
NLM_F_ACK);
if (!nlh) {
printk(KERN_ERR "netlink_pack_skb: insufficient tailroom\n");
return NULL;
}
memcpy(nlmsg_data(nlh), buff, bufflen);
return skb; /* success */
}
int netlink_init(void)
{
/* NOTE: new structure for configuring netlink socket changed in
* linux kernel 3.8
* http://stackoverflow.com/questions/15937395/netlink-kernel-create-is-not-working-with-latest-linux-kernel
*/
struct netlink_kernel_cfg nl_cfg;
nl_cfg.groups = 0; // used for multicast
nl_cfg.flags = 0; // TODO
nl_cfg.input = netlink_recv; // function that will receive data
nl_sk = netlink_kernel_create(&init_net,
NETDEV_PROTOCOL,
&nl_cfg);
if(!nl_sk) {
printk(KERN_ERR "netlink_init: error creating socket.\n");
return -1;
}
printk(KERN_INFO "netlink_init: netlink setup complete\n");
return 0;
}
void netlink_exit(void)
{
printk(KERN_INFO "netlink_exit: closing netlink\n");
/* can only be done once the process releases the socket, otherwise
* kernel will refuse to unload the module */
netlink_kernel_release(nl_sk);
}
MODULE_LICENSE("GPL");
<file_sep>/daemon/lib/helper.h
#ifndef _HELPER_H
#define _HELPER_H
#ifndef max
#define max( a, b ) ( ((a) > (b)) ? (a) : (b) )
#endif
#ifndef min
#define min( a, b ) ( ((a) < (b)) ? (a) : (b) )
#endif
#endif
<file_sep>/kernel/fo_recv.h
#ifndef _FO_RECV_H
#define _FO_RECV_H
#include "fo_comm.h"
#include "fo_access.h"
/* functions for executing file operations sent from client */
int ndfo_recv_llseek(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_read(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_write(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_aio_read(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_aio_write(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_poll(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_unlocked_ioctl(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_compat_ioctl(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_mmap(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_open(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_flush(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_release(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_fsync(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_aio_fsync(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_fasync(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_lock(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_sendpage(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_get_unmapped_area(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_check_flags(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_flock(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_splice_write(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_splice_read(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_setlease(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_fallocate(struct fo_access *acc, struct fo_req *req);
int ndfo_recv_show_fdinfo(struct fo_access *acc, struct fo_req *req);
extern int (*netdev_recv_fops[])(struct fo_access*, struct fo_req*);
#endif /* _FO_RECV_H */
| f9825a3b2f1d69082e53cd409609739b63aba904 | [
"Markdown",
"C",
"Makefile"
] | 33 | C | jakubgs/netdev | 7631eeb5a71906ff76a5ad46058df1585b31e467 | 44f9e5f433ba597f8bef5e218b5fbd3ab2958547 | |
refs/heads/main | <repo_name>kwabena-aboah/POSsystem<file_sep>/catalog/migrations/0002_auto_20201218_1301.py
# Generated by Django 3.1.1 on 2020-12-18 13:01
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('catalog', '0001_initial'),
]
operations = [
migrations.RemoveField(
model_name='product',
name='quantity',
),
migrations.AddField(
model_name='product',
name='selling_price',
field=models.DecimalField(decimal_places=2, default=1, max_digits=7),
preserve_default=False,
),
]
<file_sep>/customer/templates/customer/index.html
{% extends 'inventory/base.html' %}
{% block content %}
<div class="container">
<div class="col-md-12 col-sm-12 col-lg-12">
<h2>{{ title }}</h2>
<br>
<form action="{% url 'customer' %}" method="post" class="form">
{% csrf_token %}
{{ form.non_field_errors }}
<div class="wrapper form-control">
<label for="{{ form.name.id_for_label}}">Name</label>
{{ form.name }}<br>
<label for="{{ form.phone.id_for_label}}">Phone</label>
{{ form.phone }}<br>
<label for="{{ form.email.id_for_label}}">Email</label>
{{ form.email }}<br>
<label for="{{ form.orders.id_for_label}}">Orders</label>
{{ form.orders }}<br>
<label for="{{ form.amount_paid.id_for_label}}">Amount paid</label>
{{ form.amount_paid }}<br>
<label for="{{ form.amount_to_pay.id_for_label}}">Amount to pay</label>
{{ form.amount_to_pay }}<br>
<label for="{{ form.payment_status.id_for_label}}">Payment Status</label>
{{ form.payment_status }}<br>
<button name="submit" class="btn btn-danger">Submit</button>
</div>
</form>
</div>
</div>
{% endblock %}<file_sep>/customer/admin.py
from django.contrib import admin
from . models import Customer
from . forms import CustomerForm
class CustomerAdmin(admin.ModelAdmin):
list_display = ('id', 'name', 'phone', 'email', 'amount_paid', 'amount_to_pay', 'payment_status',)
list_filter = ('payment_status',)
search_fields = ('name',)
form = CustomerForm
list_per_page = 10
# def save_model(self, request, obj, form, change):
# recieve_customer = Customer.objects.create(id=id)
# if getattr(obj, 'created_by', True) is not None:
# obj.created_by = request.user
# obj.save()
# recieve_customer.save()
# super().save_model(request, obj, form, change)
admin.site.register(Customer, CustomerAdmin)
<file_sep>/catalog/urls.py
from django.urls import path
from django.conf.urls import url
from django.views.generic import RedirectView
from . import views
from . import apiviews
# app_name = "catalog"
urlpatterns = [
url(r'^$', RedirectView.as_view(pattern_name='order')),
path('', views.order, name='order'),
path('addition/', views.addition, name='addition'),
url(r'^order/add/(?P<product_id>[0-9A-Za-z_-]*/?$)',
views.order_add_product, name='order_add_product'),
url(r'^order/remove/(?P<product_id>[0-9]*)/?$',
views.order_remove_product, name="order_remove_product"),
url(r'^order/reset/?$', views.reset_order, name='reset_order'),
url(r'^order/amount/?$', views.order_amount, name='order_get_amount'),
url(r'^order/?$', views.order, name='order'),
url(r'^pay/momo/$', views.payment_momo, name='payment_momo'),
url(r'^pay/cash/$', views.payment_cash, name='payment_cash'),
path('cash/<int:amount>/', views.cash, name='cash'),
url(r'^view-order/(?P<order_id>[0-9]+)/?$', views.view_order,
name='view_order'),
url(r'^print-order/(?P<order_id>[0-9]+)/?$', views.print_order,
name='print_order'),
url(r'^print-current-order/?$', views.print_current_order,
name='print_current_order'),
url(r'^stock/?$', views.view_stock, name='view_stock'),
# API URL's
path('api/orders/current/', apiviews.current_order,
name='api_current_order'),
path('api/orders/current/items/', apiviews.current_order_items,
name='api_current_order_items'),
path('api/orders/current/items/<int:item_id>/',
apiviews.current_order_item,
name='api_product'),
path('api/orders/current/items/patch/<int:item_id>/',
apiviews.current_order_patch, name='api_current_order_patch'),
path('api/orders/current/items/search/<str:item_id>/',
apiviews.search_order_item, name='api_search_product'),
path('api/pay/momo/', apiviews.momo_payment, name='api_momo_payment'),
path('api/pay/cash/', apiviews.cash_payment, name='api_cash_payment'),
]
<file_sep>/catalog/tasks.py
# from celery import shared_task
from celery.task.schedules import crontab
from celery.decorators import periodic_task
from celery.utils.log import get_task_logger
import time
from datetime import timedelta, date
# import datetime
from plyer import notification
from .models import Product
logger = get_task_logger(__name__)
@periodic_task(
run_every=(crontab(minute='*/30')),
name="task_save_10_days_to_expire_product",
ignore_result=True
)
def expiry_date():
try:
products = Product.objects.all()
for product in products:
dt = date.today() + timedelta(10)
print(dt)
if product.expiring_date > dt:
logger.info("{pro} expires on {dt}. 10 days before the expiring date.".format(
pro=product.name, dt=dt))
return notification.notify(
title="URGENT INFORMATION ON PRODUCT!",
message="{pro} expires on {dt}. 10 days before the expiring date.".format(
pro=product.name, dt=dt),
app_name="JKF-VICTORY LIMITED",
ticker='URGENT PRODUCT INFORMATION',
timeout=20
)
time.sleep(15)
else:
return notification.notify(
title="INVENTORY INFORMATION!",
message="Inventory up to Date!!!",
app_name="JKF-VICTORY LIMITED",
ticker='PRODUCT INFORMATION',
timeout=20
)
time.sleep(15)
except Exception:
return 'Field "name" does not exist.'
return
@periodic_task(
run_every=(crontab(minute='*/30')),
name="task_save_expired_products",
ignore_result=True
)
def expired():
try:
products = Product.objects.all()
for product in products:
dt = date.today()
if product.expiring_date == dt:
logger.info("{pro} has expired.".format(
pro=product.name))
return notification.notify(
title="EXPIRED PRODUCT NOTIFICATION!",
message="{pro} has expired.".format(
pro=product.name),
app_name="JKF-VICTORY LIMITED",
ticker='URGENT PRODUCT INFORMATION',
timeout=15
)
time.sleep(30)
except Exception:
return 'Field "name" does not exist'
return
@periodic_task(
run_every=(crontab(minute='*/30')),
name="task_stock_level",
ignore_result=True
)
def stock_level():
try:
products = Product.objects.all()
for product in products:
if product.stock == product.minimum_stock:
logger.info("{pro} has reached it's minimum stock level.".format(
pro=product.name))
return notification.notify(
title="STOCK LEVEL NOTIFICATION!",
message="{pro} has reached it's minimum stock level.".format(
pro=product.name),
app_name="JKF-VICTORY LIMITED",
ticker='INVENTORY INFORMATION',
timeout=15
)
time.sleep(30)
except Exception:
return 'Field "name" does not exist'
return
<file_sep>/catalog/admin.py
from datetime import date
import datetime
from django.contrib import admin
from django.contrib.admin import SimpleListFilter
from django.db.models import F
from import_export import resources
from . models import Store, Supplier, Category, Product, Order, Cash, Setting
from import_export.admin import ImportExportModelAdmin
from . forms import ProductForm
admin.site.disable_action('delete_selected')
admin.site.site_header = "JKF-VICTORY LIMITED"
admin.site.site_title = "JKF-VICTORY LIMITED ADMINISTRATION"
admin.site.index_title = "JKF-VICTORY LIMITED ADMINISTRATION"
admin.site.site_url = "/"
class ProductResource(resources.ModelResource):
class Meta:
model = Product
fields = (
'name',
'price',
'selling_price',
'stock',
'stock_applies',
'minimum_stock',
'expiring_date',
'stock_level'
)
class LowStockFilter(SimpleListFilter):
title = 'Available Stock'
parameter_name = 'available_stock'
def lookups(self, request, model_admin):
return (
('low', 'Low Stock'),
('high', 'High Stock')
)
def queryset(self, request, queryset):
if self.value() == 'low':
return queryset.filter(stock__lte=F('minimum_stock'))
if self.value() == 'high':
return queryset.filter(stock__gte=F('minimum_stock'))
class ExpiredStockFilter(SimpleListFilter):
title = 'Expired Stock'
parameter_name = 'expired_stock'
def lookups(self, request, model_admin):
return (
('expired', 'Expired Product'),
)
def queryset(self, request, queryset):
if self.value() == 'expired':
return queryset.filter(expiring_date__lte=datetime.datetime.today())
class SettingAdmin(admin.ModelAdmin):
list_display = ('key', 'value')
admin.site.register(Setting, SettingAdmin)
class ProductAdmin(ImportExportModelAdmin, admin.ModelAdmin):
actions = ['delete_selected']
resource_class = ProductResource
list_display = (
'expired_field',
'name',
'price',
'selling_price',
'stock',
'stock_applies',
'minimum_stock',
'expiring_date',
'stock_level'
)
list_display_links = ('name',)
search_fileds = ('name',)
date_hierarchy = 'created_on'
form = ProductForm
list_per_page = 10
list_filter = (LowStockFilter, ExpiredStockFilter,)
def save_model(self, request, obj, form, change):
if getattr(obj, 'created_by', True) is not None:
obj.created_by = request.user
obj.save()
super().save_model(request, obj, form, change)
admin.site.register(Product, ProductAdmin)
class StoreAdmin(admin.ModelAdmin):
list_display = ('name',)
list_display_links = ('name',)
search_fileds = ('code',)
actions = ['delete_selected']
list_per_page = 5
admin.site.register(Store, StoreAdmin)
admin.site.register(Category)
class SupplierAdmin(admin.ModelAdmin):
list_display = ('name', 'email', 'tel', 'location', 'comments')
list_display_links = ('name',)
search_fileds = ('name', 'email',)
actions = ['delete_selected']
list_per_page = 10
admin.site.register(Supplier, SupplierAdmin)
# Safe deletion of orders
def safe_delete_order(modeladmin, request, queryset):
queryset.filter(done=True).delete()
safe_delete_order.short_description = "Delete completed orders"
class OrderAdmin(admin.ModelAdmin):
''' Admin site configuration for Order Model'''
# Add custom delete-if-not-done action
actions = [safe_delete_order]
# List page display configuration
list_display = ('user', 'total_price', 'done', 'last_change')
# sidebar filter configuration
list_filter = ('done',)
date_hierarchy = 'last_change'
# order by not Done
ordering = ('done',)
list_per_page = 10
admin.site.register(Order, OrderAdmin)
admin.site.register(Cash)
<file_sep>/catalog/apiviews.py
import logging
import decimal
from django.shortcuts import get_object_or_404
from django.views.decorators.csrf import csrf_exempt
from django.http import (HttpResponse, JsonResponse, HttpResponseBadRequest)
from rest_framework import status
from rest_framework.response import Response
from django.contrib import messages
from .serializers import OrderSerializer, OrderItemSerializer
from . helper import (get_current_user_order,
get_can_negative_stock, product_list_from_order)
from . models import Product, Order_Item, Order, Cash
@csrf_exempt
def current_order(request):
if not request.user.is_authenticated:
return HttpResponse(status=status.HTTP_401_UNAUTHORIZED)
order = get_current_user_order(request.user.username)
if request.method == 'DELETE':
for item in Order_Item.objects.filter(order=order):
if item.product.stock_applies:
item.product.stock += 1
item.product.save()
item.delete()
order.total_price = 0
order.save()
serializer = OrderSerializer(order)
return JsonResponse(serializer.data, safe=False)
def current_order_items(request):
if not request.user.is_authenticated:
return HttpResponse(status=status.HTTP_401_UNAUTHORIZED)
order = get_current_user_order(request.user.username)
order_items = Order_Item.objects.filter(order=order)
serializer = OrderItemSerializer(order_items, many=True)
return JsonResponse(serializer.data, safe=False)
@csrf_exempt
def current_order_item(request, item_id):
if not request.user.is_authenticated:
return HttpResponse(status=status.HTTP_401_UNAUTHORIZED)
order = get_current_user_order(request.user.username)
if request.method == 'DELETE':
order_item = get_object_or_404(Order_Item, id=item_id)
order_product = order_item.product
if order_product.stock_applies:
order_product.stock += 1
order_product.save()
order.total_price = (
order.total_price -
order_item.price).quantize(
decimal.Decimal('0.01'))
if order.total_price < 0:
logging.error("prices below 0! "
"You might be running in to the "
"10 digit total order price limit")
order.total_price = 0
order.save()
order_item.delete()
order_items = Order_Item.objects.filter(order=order)
serializer = OrderItemSerializer(order_items, many=True)
return JsonResponse(serializer.data, safe=False)
elif request.method == 'PUT':
product = get_object_or_404(Product, id=item_id)
if product.stock_applies:
if product.stock < 1 and not get_can_negative_stock():
# messages.info(request, "Insufficient stock")
return JsonResponse('{message: "Insufficient stock"}',
status=status.HTTP_400_BAD_REQUEST)
product.stock -= 1
product.save()
Order_Item.objects.create(order=order, product=product,
price=product.price, name=product.name)
# Order_Item.objects.filter(id=item_id).update(quantity=order.quantity)
order.total_price = order.get_total_cost()
order.save()
order_items = Order_Item.objects.filter(order=order)
serializer = OrderItemSerializer(order_items, many=True)
return JsonResponse(serializer.data, safe=False)
@csrf_exempt
def current_order_patch(request, item_id):
if not request.user.is_authenticated:
return HttpResponse(status=status.HTTP_401_UNAUTHORIZED)
order = get_current_user_order(request.user.username)
# new codes
if request.method == 'DELETE':
order_item = get_object_or_404(Order_Item, id=item_id)
order_product = order_item.product
if order_product.stock_applies:
order_product.stock += 1
order_product.save()
order.total_price = (
order.total_price -
order_item.price).quantize(
decimal.Decimal('0.01'))
if order.total_price < 0:
logging.error("prices below 0! "
"You might be running in to the "
"10 digit total order price limit")
order.total_price = 0
order.save()
order_item.delete()
order_items = Order_Item.objects.filter(order=order)
serializer = OrderItemSerializer(order_items, many=True)
return JsonResponse(serializer.data, safe=False)
elif request.method == 'PATCH':
# Set item_id to a count function
item_quantity = order.count_quantity()
product = get_object_or_404(Product, id=item_id)
# product.quantity = product.id
if product.stock_applies:
if product.stock < 1 and not get_can_negative_stock():
# messages.info(request, "Insufficient stock")
return JsonResponse('{message: "Insufficient stock"}',
status=status.HTTP_400_BAD_REQUEST, safe=False)
product.stock -= 1
product.save()
Order_Item.objects.update_or_create(
order=order, product=product, price=product.price, name=product.name, quantity=item_quantity)
order.total_price = order.get_total_cost()
order.save()
order_items = Order_Item.objects.filter(order=order)
serializer = OrderItemSerializer(order_items, many=True)
return JsonResponse(serializer.data, safe=False)
@csrf_exempt
def search_order_item(request, item_id):
if not request.user.is_authenticated:
return HttpResponse(status=status.HTTP_401_UNAUTHORIZED)
order = get_current_user_order(request.user.username)
if request.method == 'DELETE':
order_item = get_object_or_404(Order_Item, name=item_id)
order_product = order_item.product
if order_product.stock_applies:
order_product.stock += 1
order_product.save()
order.total_price = (
order.total_price -
order_item.price).quantize(
decimal.Decimal('0.01'))
if order.total_price < 0:
logging.error("prices below 0! "
"You might be running in to the "
"10 digit total order price limit")
order.total_price = 0
order.save()
order_item.delete()
order_items = Order_Item.objects.filter(order=order)
serializer = OrderItemSerializer(order_items, many=True)
return JsonResponse(serializer.data, safe=False)
elif request.method == 'PUT':
product = get_object_or_404(Product, name=item_id)
if product.stock_applies:
if product.stock < 1 and not get_can_negative_stock():
# messages.info(request, "Insufficient stock")
return JsonResponse('{message: "Insufficient stock"}',
status=status.HTTP_400_BAD_REQUEST)
product.stock -= 1
product.save()
Order_Item.objects.create(order=order, product=product,
price=product.price, name=product.name)
order.total_price = order.get_total_cost()
order.save()
order_items = Order_Item.objects.filter(order=order)
serializer = OrderItemSerializer(order_items, many=True)
return JsonResponse(serializer.data, safe=False)
else:
return HttpResponseBadRequest()
def cash_payment(request):
if not request.user.is_authenticated:
return HttpResponse(status=status.HTTP_401_UNAUTHORIZED)
order = get_current_user_order(request.user.username)
cash, _ = Cash.objects.get_or_create(id=0)
amount_added = 0
for product in product_list_from_order(order):
cash.amount += product.price
amount_added += product.price
cash.save()
order.done = True
order.save()
Order.objects.create(user=request.user)
return JsonResponse({'added': amount_added})
def momo_payment(request):
if not request.user.is_authenticated:
return HttpResponse(status=status.HTTP_401_UNAUTHORIZED)
order = get_current_user_order(request.user.username)
order.done = True
order.save()
Order.objects.create(user=request.user)
return HttpResponse()
<file_sep>/customer/urls.py
from django.urls import path
from django.conf.urls import url
from django.views.generic import RedirectView
from . import views
urlpatterns = [
path('', views.listCustomer, name='list-client'),
path('create/', views.createCustomer, name='customer'),
]<file_sep>/customer/migrations/0001_initial.py
# Generated by Django 3.1.1 on 2020-12-18 12:31
import customer.models
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
initial = True
dependencies = [
('catalog', '0001_initial'),
migrations.swappable_dependency(settings.AUTH_USER_MODEL),
]
operations = [
migrations.CreateModel(
name='Customer',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(max_length=200, null=True, validators=[customer.models.validate_name])),
('phone', models.CharField(blank=True, max_length=17, null=True, validators=[customer.models.validate_phone])),
('email', models.EmailField(blank=True, max_length=254, null=True)),
('amount_to_pay', models.DecimalField(decimal_places=2, default=0, max_digits=10)),
('amount_paid', models.DecimalField(decimal_places=2, default=0, max_digits=10)),
('payment_status', models.CharField(blank=True, choices=[('Paid', 'Paid'), ('Part Payment', 'Part Payment')], max_length=14, null=True)),
('date_created', models.DateTimeField(auto_now=True)),
('created_by', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to=settings.AUTH_USER_MODEL)),
('orders', models.ManyToManyField(to='catalog.Order_Item')),
],
),
]
<file_sep>/catalog/migrations/0001_initial.py
# Generated by Django 3.1.1 on 2020-12-18 12:31
import catalog.models
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
initial = True
dependencies = [
migrations.swappable_dependency(settings.AUTH_USER_MODEL),
]
operations = [
migrations.CreateModel(
name='Cash',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('amount', models.DecimalField(decimal_places=2, default=0, max_digits=7)),
],
options={
'verbose_name': 'Cash',
'verbose_name_plural': 'Cash',
},
),
migrations.CreateModel(
name='Category',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(max_length=100, validators=[catalog.models.validate_name])),
],
options={
'verbose_name': 'Category',
'verbose_name_plural': 'Categories',
'ordering': ('id',),
},
),
migrations.CreateModel(
name='Order',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('total_price', models.DecimalField(decimal_places=2, default=0, max_digits=10)),
('done', models.BooleanField(default=False)),
('last_change', models.DateTimeField(auto_now=True)),
('user', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to=settings.AUTH_USER_MODEL)),
],
),
migrations.CreateModel(
name='Setting',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('key', models.CharField(max_length=50)),
('value', models.CharField(max_length=100)),
],
),
migrations.CreateModel(
name='Store',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(max_length=150, validators=[catalog.models.validate_name])),
('description', models.TextField(blank=True, null=True)),
],
options={
'verbose_name': 'Store',
'verbose_name_plural': 'Stores',
'ordering': ('name',),
},
),
migrations.CreateModel(
name='Supplier',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(max_length=100, validators=[catalog.models.validate_name])),
('email', models.EmailField(blank=True, max_length=254)),
('tel', models.CharField(blank=True, max_length=17, validators=[catalog.models.validate_phone])),
('location', models.CharField(blank=True, max_length=200)),
('comments', models.TextField(blank=True)),
],
),
migrations.CreateModel(
name='Product',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(max_length=200, validators=[catalog.models.validate_name])),
('price', models.DecimalField(decimal_places=2, max_digits=7)),
('stock_applies', models.BooleanField()),
('quantity', models.IntegerField(default=1, help_text='Number of items to purchase. Only update this field at the sales page for clients buying more than 1 items.')),
('minimum_stock', models.PositiveSmallIntegerField(default=0)),
('stock', models.IntegerField(default=0)),
('expiring_date', models.DateField()),
('colour', models.CharField(choices=[('blue', 'Blue'), ('green', 'Green'), ('yellow', 'Yellow'), ('orange', 'Orange'), ('purple', 'Purple'), ('black', 'Black'), ('pink', 'Pink'), ('cyan', 'Cyan')], default='blue', max_length=10)),
('created_on', models.DateTimeField(auto_now_add=True)),
('category', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='product_category', to='catalog.category')),
('created_by', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to=settings.AUTH_USER_MODEL)),
('store', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='product_store', to='catalog.store')),
('supplier', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='product_supplier', to='catalog.supplier')),
],
options={
'verbose_name': 'Product',
'verbose_name_plural': 'Products',
'ordering': ('name',),
},
),
migrations.CreateModel(
name='Order_Item',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('price', models.DecimalField(decimal_places=2, max_digits=7)),
('quantity', models.IntegerField(default=1)),
('name', models.CharField(max_length=100)),
('timestamp', models.DateTimeField(auto_now=True)),
('order', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='items', to='catalog.order')),
('product', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='catalog.product')),
],
),
]
<file_sep>/catalog/templates/inventory/index.html
{% extends 'inventory/base.html' %}
{% block content %}
<div class="container-fluid">
<div class="row">
<div class="col-sm-6">
<div class="row">
{% if list %}
{% for product in list %}
<div class="col-sm-6 col-md-4" style="padding-top: 5px; padding-bottom: 5px;">
<a role="button" class="btn btn-primary btn-block {{ product.colour }}"
href="#"
onclick="app.addProduct({{ product.id }});">
{{ product.name }}<br>{{ currency }}{{ product.price }}
</a>
</div>
{% endfor %}
{% endif %}
</div>
</div>
<div class="col-sm-6">
<table class="table">
<thead>
<tr>
<th scope="col">Name</th>
<th scope="col">Price</th>
<th scope="col">Quantity</th>
</tr>
</thead>
<tbody>
<tr v-for="item in items" :key="item.id">
<td>[[ item.name ]]</td>
<td>[[ item.price ]]</td>
<td data-th="Quantity">
<input type="number" class="form-control" v-model.number="item.quantity"
@blur="apdateProduct(item.quantity)"
@change="apdateProduct(item.quantity)"
step="1"
min="1"
max="99"/>
</td>
<td><button class="btn btn-danger" @click="removeProduct(item.id)">X</button></td>
</tr>
<tr>
<th scope="row">Total</th>
<td>[[ order.total_price ]]</td>
<td></td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
{% endblock %}<file_sep>/catalog/forms.py
from django import forms
from . models import Product # , Order_Item
class ProductForm(forms.ModelForm):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
class Meta:
model = Product
fields = ('name', 'price', 'selling_price', 'stock_applies', 'minimum_stock',
'stock', 'expiring_date', 'supplier', 'store', 'category', 'colour')
# class Order_ItemForm(forms.ModelForm):
# class Meta:
# model = Order_Item
# fields = ['quantity']
<file_sep>/summary/models.py
from django.db import models
from catalog.models import Order, Order_Item
class OrderSummary(Order):
class Meta:
proxy = True
verbose_name = 'Order Report'
verbose_name_plural = 'Order Reports'
class OrderItemSummary(Order_Item):
class Meta:
proxy = True
verbose_name = "Product Report"
verbose_name_plural = "Product Reports"
<file_sep>/README.md
POSsystem based on EpPOS system
<file_sep>/catalog/models.py
import re
import time
from datetime import date, timedelta
from django.db import models
from django.dispatch import receiver
from plyer import notification
from django.utils.html import format_html
from django.contrib.auth.models import User
from django.core.exceptions import ValidationError
from django.core.validators import RegexValidator
from django.urls import reverse
def validate_name(names):
regex_string = r'^\w[\w ]*$'
search = re.compile(regex_string).search
result = bool(search(names))
if not result:
raise ValidationError("Please only use letters, "
"numbers and underscores or spaces."
"The name cannot start with space.")
def validate_phone(phone):
phone_regex = r'^\+?1?\d{9,15}$'
search = re.compile(phone_regex).search
result = bool(search(phone))
if not result:
raise RegexValidator("Phone numbers must be entered in the format:"
"'+999999999.' Up to 15 digits allowed.")
class Store(models.Model):
"""This class manages Store divisions, warehouses, diffrent branches etc. """
name = models.CharField(max_length=150, validators=[validate_name])
description = models.TextField(blank=True, null=True)
class Meta:
ordering = ('name',)
verbose_name = 'Store'
verbose_name_plural = 'Stores'
def __str__(self):
return self.name
class Supplier(models.Model):
"""This class manages the suppliers of the products"""
name = models.CharField(max_length=100, validators=[validate_name])
email = models.EmailField(blank=True)
tel = models.CharField(max_length=17, validators=[
validate_phone], blank=True)
location = models.CharField(max_length=200, blank=True)
comments = models.TextField(blank=True)
def __str__(self):
return self.name
class Category(models.Model):
name = models.CharField(max_length=100, validators=[validate_name])
def __str__(self):
return self.name
class Meta:
ordering = ('id',)
verbose_name = 'Category'
verbose_name_plural = 'Categories'
class Product(models.Model):
""" This class manages information related to the products."""
# The different colours, to use as Product.<colour>
BLUE = "blue"
GREEN = "green"
YELLOW = "yellow"
ORANGE = "orange"
PURPLE = "purple"
BLACK = "black"
PINK = "pink"
CYAN = "cyan"
# Colour choices
COLOUR_CHOICES = (
(BLUE, "Blue"),
(GREEN, "Green"),
(YELLOW, "Yellow"),
(ORANGE, "Orange"),
(PURPLE, "Purple"),
(BLACK, "Black"),
(PINK, "Pink"),
(CYAN, "Cyan"),
)
name = models.CharField(max_length=200, validators=[validate_name])
price = models.DecimalField(max_digits=7, decimal_places=2)
stock_applies = models.BooleanField()
selling_price = models.DecimalField(max_digits=7, decimal_places=2)
minimum_stock = models.PositiveSmallIntegerField(default=0)
stock = models.IntegerField(default=0)
expiring_date = models.DateField()
supplier = models.ForeignKey(
Supplier, on_delete=models.CASCADE, blank=True, null=True, related_name='product_supplier')
store = models.ForeignKey(
Store, on_delete=models.CASCADE, blank=True, null=True, related_name="product_store")
category = models.ForeignKey(
Category, on_delete=models.CASCADE, blank=True, null=True, related_name="product_category")
colour = models.CharField(
max_length=10, choices=COLOUR_CHOICES, default="blue")
created_on = models.DateTimeField(auto_now_add=True)
created_by = models.ForeignKey(User, on_delete=models.CASCADE)
class Meta:
ordering = ('name',)
verbose_name = 'Product'
verbose_name_plural = 'Products'
def __str__(self):
return self.name
def expired_field(self):
if date.today() >= self.expiring_date:
return format_html(u'<span style="color: red;">Expired</span>')
expired_field.allow_tags = True
def stock_level(self):
if self.stock <= self.minimum_stock:
return format_html(u"<span style='color: red;'>Low</span>")
else:
return format_html(u"<span style='color: green;'>High</span>")
stock_level.allow_tags = True
class Order(models.Model):
user = models.ForeignKey(User, on_delete=models.CASCADE)
total_price = models.DecimalField(
max_digits=10, decimal_places=2, default=0)
done = models.BooleanField(default=False)
last_change = models.DateTimeField(auto_now=True)
def get_absolute_url(self):
return reverse('view_order', args=[self.id])
def get_total_cost(self):
""" Calculate total order cost for orders"""
return sum(item.get_cost() for item in self.items.all())
def count_quantity(self):
""" Count for the quantity of order_items needed by customer."""
result = 0
for item in self.items.all():
result += 1 * item.quantity
return result
class Cash(models.Model):
amount = models.DecimalField(max_digits=7, decimal_places=2, default=0)
def __str__(self):
return self.amount
class Meta:
verbose_name = 'Cash'
verbose_name_plural = 'Cash'
class Order_Item(models.Model):
product = models.ForeignKey(Product, on_delete=models.CASCADE)
order = models.ForeignKey(
Order, related_name='items', on_delete=models.CASCADE)
price = models.DecimalField(max_digits=7, decimal_places=2)
quantity = models.IntegerField(default=1)
name = models.CharField(max_length=100)
timestamp = models.DateTimeField(auto_now=True)
def __str__(self):
return self.product.name
def get_cost(self):
return self.price * self.quantity
class Setting(models.Model):
key = models.CharField(max_length=50)
value = models.CharField(max_length=100)
def __str__(self):
return self.key
def __bool__(self):
return bool(self.value)
__nonzero__ = __bool__
<file_sep>/summary/templatetags/mathtags.py
from django import template
register = template.Library()
@register.filter()
def percentof(n1, n2):
try:
return (float(n1) / float(n2)) * 100
except (ZeroDivisionError, TypeError):
return None
# return '{0:.2%}'.format(value)
<file_sep>/summary/admin.py
from django.contrib import admin
from django.db.models import Count, Sum, Min, Max, DateTimeField
from django.db.models.functions import Trunc
from . import models
def get_next_in_date_hierarchy(request, date_hierarchy):
if date_hierarchy + '__day' in request.GET:
return 'hour'
if date_hierarchy + '__month' in request.GET:
return 'day'
if date_hierarchy + '__year' in request.GET:
return 'week'
return 'month'
@admin.register(models.OrderSummary)
class LoadOrderSummaryAdmin(admin.ModelAdmin):
change_list_template = 'admin/order_change_list.html'
actions = None
date_hierarchy = 'last_change'
show_full_result_count = False
list_filter = (
'user__username',
)
def has_add_permission(self, request):
return False
def has_delete_permission(self, request, obj=None):
return False
def has_change_permission(self, request, obj=None):
return True
def has_module_permission(self, request):
return True
def changelist_view(self, request, extra_context=None):
response = super().changelist_view(request, extra_context=extra_context)
try:
qs = response.context_data['cl'].queryset
except (AttributeError, KeyError):
return response
# list view
metrics = {
'total': Count('id'),
'total_cost': Sum('total_price')
}
response.context_data['summary'] = list(
qs
.values('id', 'total_price')
.annotate(**metrics)
.order_by('-last_change')
)
# list view summary
response.context_data['summary_total'] = dict(qs.aggregate(**metrics))
# Chart
period = get_next_in_date_hierarchy(request, self.date_hierarchy)
response.context_data['period'] = period
summary_over_time = qs.annotate(
period=Trunc('last_change', 'day', output_field=DateTimeField()),
).values('id').annotate(total=Sum('total_price')).order_by('last_change')
summary_range = summary_over_time.aggregate(
low=Min('total'),
high=Max('total'),
)
high = summary_range.get('high', 0)
low = summary_range.get('low', 0)
response.context_data['summary_over_time'] = [{
'period': x['id'],
'total': x['total'] or 0,
'pct': ((x['total'] or 0) - low) / (high - low) * 100
if high > low else 0,
} for x in summary_over_time]
return response
@admin.register(models.OrderItemSummary)
class LoadOrderItemSummaryAdmin(admin.ModelAdmin):
change_list_template = 'admin/orderitem_change_list.html'
actions = None
date_hierarchy = 'timestamp'
show_full_result_count = False
def has_add_permission(self, request):
return False
def has_delete_permission(self, request, obj=None):
return False
def has_change_permission(self, request, obj=None):
return True
def has_module_permission(self, request):
return True
def changelist_view(self, request, extra_context=None):
response = super().changelist_view(request, extra_context=extra_context)
try:
qs = response.context_data['cl'].queryset
except (AttributeError, KeyError):
return response
# list view
metrics = {
'total_product': Count('product__name'),
'total_price': Sum('price')
}
response.context_data['summary'] = list(
qs
.values('product__name', 'price')
.annotate(**metrics)
.order_by('-timestamp')
)
# list view summary
response.context_data['summary_total'] = dict(qs.aggregate(**metrics))
# Chart
period = get_next_in_date_hierarchy(request, self.date_hierarchy)
response.context_data['period'] = period
summary_over_time = qs.annotate(
period=Trunc('timestamp', 'day', output_field=DateTimeField()),
).values('product__name').annotate(total=Sum('price')).order_by('timestamp')
summary_range = summary_over_time.aggregate(
low=Min('total'),
high=Max('total'),
)
high = summary_range.get('high', 0)
low = summary_range.get('low', 0)
response.context_data['summary_over_time'] = [{
'period': x['product__name'],
'total': x['total'] or 0,
'pct': ((x['total'] or 0) - low) / (high - low) * 100
if high > low else 0,
} for x in summary_over_time]
return response
<file_sep>/customer/views.py
from django.shortcuts import render, redirect
from django.http import HttpResponseRedirect, Http404, HttpResponse
from django.urls import reverse
from django.contrib import messages
from django.contrib.auth.decorators import login_required
from .models import Customer
# from catalog import helper
from . forms import CustomerForm
@login_required
def createCustomer(request):
if request.method != 'POST':
form = CustomerForm()
else:
form = CustomerForm(request.POST)
if form.is_valid():
new_customer = form.save(commit=False)
new_customer.created_by = request.user
new_customer.save()
messages.success(request, "Record successfully added!")
return redirect('/')
context = {'form': form, 'title': 'Create Customer'}
return render(request, 'customer/index.html', context)
@login_required
def listCustomer(request):
customers = Customer.objects.all()
title = "Manage Creditors"
context = {'customers': customers, 'title': title}
return render(request, 'customer/creditor.html', context)
<file_sep>/catalog/views.py
import logging
import decimal
from django.core.exceptions import ObjectDoesNotExist
from django.shortcuts import render, get_object_or_404#, redirect
from django.http import (HttpResponse, HttpResponseForbidden,
HttpResponseBadRequest, HttpResponseRedirect)
from django.urls import reverse
from django.contrib import messages
from django.contrib.auth import authenticate
from django.contrib.auth.models import User
from django.contrib.auth import login as auth_login
from django.contrib.auth.decorators import login_required
from django.contrib.auth.decorators import user_passes_test
from . models import Product, Order, Cash, Order_Item
from . tasks import expiry_date, expired, stock_level
from . import helper
def login(request):
if request.method == 'POST':
username = request.POST.get('username')
password = request.POST.get('<PASSWORD>')
user = authenticate(username=username, password=password)
if user:
if user.is_active:
messages.success(request, "Login Successful")
auth_login(request, user)
return HttpResponseRedirect(reverse('order'))
else:
return messages.info(request, "Your account was inactive.")
else:
print("Someone tried to login and failed.")
print("They used username: {} and password: {}".format(
username, password))
messages.error(request, "Invalid login details given")
return HttpResponseRedirect('/')
else:
return render(request, 'registration/login.html', {'title': 'LOGIN PAGE'})
@login_required
def order(request):
# Sort products by code, then by name
list = Product.objects.order_by('name')
currency = helper.get_currency()
expiry_day = expiry_date()
expired_today = expired()
stock_level_info = stock_level()
context = {
'list': list,
'currency': currency,
'expiry_day': expiry_day,
'expired_today': expired_today,
'stock_level_info': stock_level_info,
'title': 'Orders',
}
return render(request, 'inventory/index.html', context=context)
def _addition_no_stock(request):
cash, current_order, currency = helper.setup_handling(request)
total_price = current_order.total_price
list = Order_Item.objects.filter(order=current_order)
context = {
'list': list,
'total_price': total_price,
'cash': cash,
'succesfully_payed': False,
'payment_error': False,
'amount_added': 0,
'currency': currency,
'stock_error': True,
}
return render(request, 'inventory/addition.html', context=context)
@login_required
def addition(request):
cash, current_order, currency = helper.setup_handling(request)
total_price = current_order.total_price
list = Order_Item.objects.filter(order=current_order)
context = {
'list': list,
'total_price': total_price,
'cash': cash,
'succesfully_payed': False,
'payment_error': False,
'amount_added': 0,
'currency': currency,
'stock_error': False,
}
return render(request, 'inventory/addition.html', context=context)
def _show_order(request, order_id, should_print):
currency = helper.get_currency()
order = get_object_or_404(Order, id=order_id)
items = Order_Item.objects.filter(order=order)
company = helper.get_company()
context = {
'list': items,
'order': order,
'currency': currency,
'company': company,
'print': should_print
}
return render(request, 'inventory/view_order.html', context=context)
@login_required
def view_order(request, order_id):
return _show_order(request, order_id, False)
@login_required
def print_order(request, order_id):
return _show_order(request, order_id, True)
@login_required
def print_current_order(request):
# Get the user. This is quite a roundabout, sorry
usr = User.objects.get_by_natural_key(request.user.username)
q = Order.objects.filter(user=usr)\
.order_by('-last_change')
# This is an edge case that would pretty much never occur
if q.count() < 1:
return HttpResponseBadRequest
return _show_order(request, q[0].id, True)
@login_required
def order_amount(request):
_, current_order, _ = helper.setup_handling(request)
return HttpResponse(current_order.total_price)
@login_required
def order_add_product(request, product_id):
_, current_order, _ = helper.setup_handling(request)
if product_id.isdigit():
try:
to_add = Product.objects.get(id=product_id)
except ObjectDoesNotExist:
to_add = get_object_or_404(Product, code=product_id)
else:
to_add = get_object_or_404(Product, code=product_id)
# Make sure we can't go under 0 stock
if to_add.stock_applies:
if to_add.stock < 1 and not helper.get_can_negative_stock():
return _addition_no_stock(request)
to_add.stock -= 1
to_add.save()
Order_Item.objects.create(order=current_order, product=to_add,
price=to_add.price, name=to_add.name)
current_order.total_price = current_order.get_total_cost()
current_order.save()
return addition(request)
@login_required
def order_remove_product(request, product_id):
cash, current_order, _ = helper.setup_handling(request)
order_item = get_object_or_404(Order_Item, id=product_id)
order_product = order_item.product
if order_product.stock_applies:
order_product.stock += 1
order_product.save()
current_order.total_price = current_order.get_total_cost()
if current_order.total_price < 0:
logging.error("prices below 0! "
"You might be running in to the "
"10 digit total order price limit")
current_order.total_price = 0
current_order.save()
order_item.delete()
# I only need default values.
return addition(request)
@login_required
@user_passes_test(lambda u: u.is_superuser)
def reset_order(request):
cash, current_order, _ = helper.setup_handling(request)
for item in Order_Item.objects.filter(order=current_order):
if item.product.stock_applies:
item.product.stock += item.product.quantity
item.product.save()
item.delete()
current_order.total_price = 0
current_order.save()
# I just need default values. Quite useful
return addition(request)
@login_required
def payment_cash(request):
succesfully_payed = False
payment_error = False
amount_added = 0
cash, current_order, currency = helper.setup_handling(request)
for product in helper.product_list_from_order(current_order):
cash.amount += product.price * product.quantity
amount_added += product.price * product.quantity
cash.save()
current_order.done = True
current_order.save()
current_order = Order.objects.create(user=request.user)
succesfully_payed = True
total_price = current_order.total_price
list = Order_Item.objects.filter(order=current_order)
context = {
'list': list,
'total_price': total_price,
'cash': cash,
'succesfully_payed': succesfully_payed,
'payment_error': payment_error,
'amount_added': amount_added,
'currency': currency,
}
return render(request, 'inventory/addition.html', context=context)
@login_required
def payment_momo(request):
succesfully_payed = False
payment_error = False
cash, current_order, currency = helper.setup_handling(request)
current_order.done = True
current_order.save()
current_order = Order.objects.create(user=request.user)
succesfully_payed = True
total_price = current_order.total_price
list = Order_Item.objects.filter(order=current_order)
context = {
'list': list,
'total_price': total_price,
'cash': cash,
'succesfully_payed': succesfully_payed,
'payment_error': payment_error,
'amount_added': 0,
'currency': currency,
}
return render(request, 'inventory/addition.html', context=context)
# We can't use the `@login_required` decorator here because this
# page is never shown to the user and only used in AJAX requests
def cash(request, amount):
if (request.user.is_authenticated):
cash, _ = Cash.objects.get_or_create(id=0)
cash.amount = amount
cash.save()
return HttpResponse('')
else:
return HttpResponseForbidden('403 Forbidden')
@login_required
@user_passes_test(lambda u: u.is_superuser)
def view_stock(request):
stock_products = Product.objects.filter(stock_applies=True)
company = helper.get_company()
context = {
'list': stock_products,
'company': company
}
return render(request, 'inventory/stock.html', context=context)
<file_sep>/customer/models.py
import re
from django.core.exceptions import ValidationError
from django.core.validators import RegexValidator
from django.db import models
from catalog.models import Order_Item
from django.contrib.auth.models import User
def validate_name(names):
regex_string = r'^\w[\w ]*$'
search = re.compile(regex_string).search
result = bool(search(names))
if not result:
raise ValidationError("Please only use letters, "
"numbers and underscores or spaces."
"The name cannot start with space.")
def validate_phone(phone):
phone_regex = r'^\+?1?\d{9,15}$'
search = re.compile(phone_regex).search
result = bool(search(phone))
if not result:
raise RegexValidator("Phone numbers must be entered in the format:"
"'+999999999.' Up to 15 digits allowed.")
class Customer(models.Model):
PAID = 'Paid'
PART = 'Part Payment'
STATUS = (
(PAID, 'Paid'),
(PART, 'Part Payment'),
)
name = models.CharField(max_length=200, null=True,
validators=[validate_name])
phone = models.CharField(max_length=17, null=True,
validators=[validate_phone], blank=True)
email = models.EmailField(blank=True, null=True)
orders = models.ManyToManyField(Order_Item)
amount_to_pay = models.DecimalField(max_digits=10, decimal_places=2, default=0)
amount_paid = models.DecimalField(
max_digits=10, decimal_places=2, default=0)
payment_status = models.CharField(
max_length=14, choices=STATUS, blank=True, null=True)
created_by = models.ForeignKey(User, on_delete=models.CASCADE)
date_created = models.DateTimeField(auto_now=True)
def __str__(self):
return self.name
# def save(self, *args, **kwargs):
# for order in self.orders:
# if self.amount == order.price:
# return self.payment_status.Paid
# else:
# return self.payment_status.PART
# return super(Customer, self).save(*args, **kwargs)
<file_sep>/staticfiles/js/app.js
$(document).ready(function() {
$('#resetcashpopover').popover({html:true});
});
$('#CodeModal').on('shown.bs.modal', function (e) {
$('#codeInput').focus();
})
var app = new Vue({
delimiters: ['[[', ']]'],
el: '#app',
data: {
order: {},
items: [],
cashgot: 0.0
},
methods: {
update_order: function() {
fetch("/api/orders/current/")
.then(res => res.json())
.then((out) => {
this.order = out;
});
},
update_items: function() {
fetch("/api/orders/current/items/")
.then(res => res.json())
.then((out) => {
this.items = out;
});
},
cashmodal: function() {
$('#cashgot').focus();
console.log(this.order.total_price);
document.getElementById('topay').value = this.order.total_price;
},
removeProduct: function(product_id) {
fetch("/api/orders/current/items/" + encodeURIComponent(product_id) + "/", {method: 'DELETE'})
.then(response => {
if (response.status == 200) {
response.json().then(json => {
this.items = json;
});
this.update_order();
} else {
window.alert("Can't remove product");
console.log(response);
}
});
},
addProduct: function(product_id) {
fetch("/api/orders/current/items/" + encodeURIComponent(product_id) + "/", {method: 'PUT'})
.then(response => {
if (response.status == 404) {
window.alert("Product not found");
} else if (response.status != 200) {
console.log(response);
window.alert("Product out of stock. Refill please!");
} else {
this.update_order();
// this must be a promise
response.json().then(json => {
this.items = json;
});
}
});
},
resetCash: function() {
var value = document.getElementById('resetcashinput').value;
fetch("/cash/" + encodeURIComponent(value))
.then(response => {
if (response.status != 200) {
window.alert("Can't reset cash");
console.log(response);
} else {
$('#cashresetamount').text(encodeURIComponent(value));
$('#cashresetdiv').show()
$('#resetcashpopover').popover('hide');
}
});
},
payed: function(method) {
if (confirm("Do you want to print the addition?")) {
console.log(this.order.id);
window.open("/print-order/" + this.order.id, "_blank").focus();
}
if (method == "cash") {
fetch("/api/pay/cash/").then(response => {
if (response.status != 200) {
window.alert("Couldn't pay");
console.log(response);
} else {
response.json().then(json => {
window.alert("Added " + json.added + " to the register");
});
this.update_order();
this.update_items();
}
});
} else if (method == "momo") {
if (!confirm('Are you sure?'))
return;
fetch("/api/pay/momo/").then(response => {
if (response.status != 200) {
window.alert("Couldn't pay");
console.log(response);
} else {
window.alert("Successfully paid.");
this.update_order();
this.update_items();
}
});
}
console.log("Person payed");
},
scanCode: function() {
this.addProduct(document.getElementById('codeInput').value);
document.getElementById('codeInput').value = "";
document.getElementById('codeInput').focus();
},
reset: function() {
fetch("/api/orders/current/", {method: 'delete'})
.then(response => {
if (response.status == 200) {
window.alert("Order cleared");
} else {
window.alert("Can't clear order");
console.log(response);
}
});
this.update_order();
this.update_items();
}
},
created() {
this.update_order();
this.update_items();
}
});
$('#cashModal').on('shown.bs.modal', function() {
app.cashmodal();
});
$(document).ready(function() {
// This shallst load /addition in its div
$('#resetcashpopover').popover({html:true});
});
$('#CodeModal').on('shown.bs.modal', function (e) {
$('#codeInput').focus();
})<file_sep>/Project/urls.py
"""Project URL Configuration
The `urlpatterns` list routes URLs to views. For more information please see:
https://docs.djangoproject.com/en/3.1/topics/http/urls/
Examples:
Function views
1. Add an import: from my_app import views
2. Add a URL to urlpatterns: path('', views.home, name='home')
Class-based views
1. Add an import: from other_app.views import Home
2. Add a URL to urlpatterns: path('', Home.as_view(), name='home')
Including another URLconf
1. Import the include() function: from django.urls import include, path
2. Add a URL to urlpatterns: path('blog/', include('blog.urls'))
"""
from django.contrib import admin
from django.contrib.auth import views as auth_views
from django.views.generic.base import RedirectView
from catalog.views import login
from django.urls import path, re_path, include
from django.conf import settings
from django.conf.urls import url
from django.contrib.staticfiles.urls import staticfiles_urlpatterns
from django.conf.urls.static import static
from django.views.static import serve
urlpatterns = [
re_path(r'^admin/', admin.site.urls),
# path('', RedirectView.as_view(url='/', permanent=True)),
path('', include('catalog.urls')),
path('customers/', include('customer.urls')),
path('accounts/logout/', auth_views.LogoutView.as_view(), {'next_page': '/'}, name='logout'),
path('accounts/login/', login, name='login'),
path('accounts/', include('django.contrib.auth.urls')),
path('api-auth/', include('rest_framework.urls')),
url(r'^static/(?P<path>.*)$', serve,
{'document_root': settings.STATIC_ROOT}),
] + static(settings.STATIC_URL, document_root=settings.STATIC_ROOT)
urlpatterns += staticfiles_urlpatterns()
| 98b6f02df14f420161f7067f6adf1f6217f1a940 | [
"Markdown",
"Python",
"JavaScript",
"HTML"
] | 22 | Python | kwabena-aboah/POSsystem | 727ba603fb1a18cf8ebcc802c257330c85e93302 | 3e0588c289f3afbaa546e45d81acdc6ba23c264d | |
refs/heads/master | <repo_name>project-pantheon/pantheon_glob_planner<file_sep>/pantheon_planner/plots_functions.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Tue Jan 8 14:23:33 2019
@author: Jacopo
"""
import os
import matplotlib.pyplot as plt
def getCurrWDir():
return os.getcwd()
def plotMapTrees(trees_pos,tree_radious):
plt.figure(figsize=(8,6))
plt.scatter(trees_pos[:,0],trees_pos[:,1],color='g',s=100 * tree_radious)
for i, txt in enumerate(trees_pos[:,2]):
plt.annotate(txt, (trees_pos[i,0],trees_pos[i,1]))
plt.axis('equal')
plt.title("Trees positions")
plt.show()
def plotCapturePts(capture_pos):
plt.figure(figsize=(8,6))
plt.scatter(capture_pos[:,0],capture_pos[:,1],color='b')
plt.axis('equal')
plt.title("Captures points positions")
plt.show()
def plotTreesAndCaptures(trees_pos,capture_pos,tree_radious):
plt.figure(figsize=(8,6))
ax = plt.gca()
ax.cla()
# plt.scatter(trees_pos[:,0],trees_pos[:,1],color='g', s = 100 * tree_radious)
plt.scatter(capture_pos[:,0],capture_pos[:,1],color='b')
for i, txt in enumerate(trees_pos[:,2]):
circle3 = plt.Circle((trees_pos[i,0], trees_pos[i,1]), tree_radious, color='g', clip_on=False)
plt.annotate(int(txt), (trees_pos[i,0],trees_pos[i,1]))
ax.add_artist(circle3)
for i, txt in enumerate(capture_pos[:,0]):
plt.annotate(str(i+1), (capture_pos[i,0],capture_pos[i,1]))
plt.axis('equal')
plt.title("All the points")
name = "capture_pts_and_trees"
path = getCurrWDir()
plt.savefig( path + "/results/"+ name, dpi = 800 ,format = 'pdf')
plt.show()
def plotPoints(points,edges):
x = points[:,0].flatten()
y = points[:,1].flatten()
fig, ax= plt.subplots(figsize=(10,8))
#ax.scatter(points[:,0],points[:,1])
num =range(points.shape[0])
for i,txt in enumerate(num):
ax.annotate(txt, (points[i,0], points[i,1]),)
plt.plot(x[edges.T], y[edges.T], linestyle='-', color='y')
def plotWithoutLines(points,centers,n_col,n_row):
x = points[:,0].flatten()
y = points[:,1].flatten()
plt.figure(figsize=(16,12))
plt.plot(centers[:,0],centers[:,1],'o',color ='g')
plt.plot(x,y,'o',color ='r')
name = "points "+str(n_col)+"x"+str(n_row)
plt.savefig("/home/majo/libs/pyconcorde/pantheon_planner/results/"+name, dpi = 800 ,format = 'pdf')
def plotSolutionGraph(capture_pos,edges,trees_pos,filename):
x = capture_pos[:,0]
y = capture_pos[:,1]
plt.figure(figsize=(8,6))
ax = plt.gca()
ax.cla()
plt.scatter(capture_pos[:,0],capture_pos[:,1],color='b')
for i, txt in enumerate(trees_pos[:,2]):
circles = plt.Circle((trees_pos[i,0], trees_pos[i,1]), 0.3, color='g', clip_on=False)
plt.annotate(int(txt), (trees_pos[i,0],trees_pos[i,1]))
ax.add_artist(circles)
for i, txt in enumerate(capture_pos[:,0]):
plt.annotate(str(i+1), (capture_pos[i,0],capture_pos[i,1]))
plt.plot(x[edges.T], y[edges.T], linestyle='-', color='r')
plt.annotate("start/stop", (x[edges[0,0]]-0.5,y[edges[0,0]]-0.5))
path = getCurrWDir()
plt.savefig(path + "/results/" + filename, format = 'pdf')
plt.axis("equal")
plt.show()
def plotGraph(points,edges,trees_pos,n_col,n_row):
x = points[:,0].flatten()
y = points[:,1].flatten()
plt.figure(figsize=(8,6))
ax = plt.gca()
ax.cla()
# plt.figure(figsize=(12,8))
# plt.plot(trees_pos[:,0],trees_pos[:,1],'o',color ='g')
for i, txt in enumerate(trees_pos[:,2]):
circles = plt.Circle((trees_pos[i,0], trees_pos[i,1]), 0.3, color='g', clip_on=False)
plt.annotate(txt, (trees_pos[i,0],trees_pos[i,1]))
ax.add_artist(circles)
plt.plot(x[edges.T], y[edges.T], linestyle='-', color='y',markerfacecolor='red', marker='o')
plt.plot(x,y,'o',color ='r')
plt.plot(x[edges.T], y[edges.T], color='y',markerfacecolor='red', marker='o')
name = str(n_col)+"x"+str(n_row)
path = getCurrWDir()
plt.savefig(path + "/results/" + name, format = 'pdf')
plt.axis("equal")
plt.show()
def plotGraphLight(points,edges,centers):
x = points[:,0].flatten()
y = points[:,1].flatten()
plt.figure(figsize=(10,8))
plt.plot(centers[:,0],centers[:,1],'o',color ='g')
plt.plot(x[edges.T], y[edges.T], linestyle='-', color='y',markerfacecolor='red', marker='o')
<file_sep>/README.md
# pantheon_glob_planner
This repository contains the global planner for the sherpa robots of the Pantheon Project.
-----
It includes also the [PyConcorde](https://github.com/jvkersch/pyconcorde) which acts as a Python wrapper around the [Concorde TSP
solver] (http://www.math.uwaterloo.ca/tsp/concorde.html).
<file_sep>/pantheon_planner/run_test.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
import numpy as np
from concorde.tsp import TSPSolver
import functions as fc
import plots_functions as pf
import file_reader_writer as frw
import time
import sys
import os
from shapely.geometry import LineString
from shapely.geometry import Point
from termcolor import colored
from collections import defaultdict
from concorde.tests.data_utils import get_dataset_path
np.set_printoptions(threshold=sys.maxsize)
def getCurrWDir():
return os.getcwd()
def computeTsp(filename):
solver = TSPSolver.from_tspfile( getCurrWDir() + "/inputs/" + filename)
start_time = time.time()
solution = solver.solve()
eval_time = time.time() - start_time
print( colored("--- %s seconds ---" % eval_time) )
print( colored("Outcome TSP:","red") )
print(solution.found_tour)
print( colored("With optimal value [m]:","red") )
print(solution.optimal_value/100)
return solution,eval_time
def distMatrix(n_trees, points,centers): #ASYMMETRIC MATRIX
dist = np.zeros(shape=[n_trees*4,n_trees*4],dtype=int)
for i in range(n_trees*4):
for j in range(n_trees*4):
if ((i%4 == 0) and (j == i+3)) or ((i%4 == 1)and(j ==i+1)):
dist[i][j] = 1000000
dist[j][i] = 1000000
else:
dist[i][j] = np.round(np.sqrt(np.power(points[i][0]-points[j][0],2)+np.power(points[i][1]-points[j][1],2)))
return dist
def computeAdjMatrix(n_rows,n_cols,inBetweenRows):
#given the n° of trees we have distribution based on inBetweenRows
if ( inBetweenRows == 1):
MAP_DIM = ( n_rows + 1 ) * ( n_cols + 1 )
BLOCK_SIZE = ( n_cols + 1 );
N_BLOCKS = ( n_rows + 1) ;
v1 = np.ones( (MAP_DIM - BLOCK_SIZE) );
COLS_connections = np.add( np.diag(v1,BLOCK_SIZE), np.diag(v1,-BLOCK_SIZE) );
v2 = np.ones( (BLOCK_SIZE-1) );
BLOCK = np.add( np.diag(v2,1), np.diag(v2,-1) );
ROWS_connections = np.zeros((MAP_DIM,MAP_DIM))
for i in range(N_BLOCKS):
ROWS_connections[ i*BLOCK_SIZE : (i+1) * BLOCK_SIZE, i*BLOCK_SIZE : (i+1) * BLOCK_SIZE] = BLOCK;
Adj_matrix = np.add( COLS_connections, ROWS_connections );
else:
MAP_DIM = ( ( n_rows + 1) * n_cols ) + ( ( n_cols + 1) * n_rows )
v1 = np.ones( (MAP_DIM - n_cols) );
v2 = np.ones( (MAP_DIM - (n_cols + 1) ) );
for i in range(1,n_rows+1):
v1[ n_cols + (i-1)*(2*n_cols +1) ] = 0;
for i in range(1,n_rows):
v2[2*n_cols+ (i-1)*(2*n_cols +1)] = 0
upper = np.add( np.diag(v1,n_cols) , np.diag(v2, n_cols + 1) )
lower = np.transpose(upper)
Adj_matrix = np.add(upper,lower)
return Adj_matrix
def fromMatrix2List(A):
graph = defaultdict(list)
edges = set()
# graph2 = defaultdict(list)
# edges2 = set()
for i, v in enumerate(A, 1):
for j, u in enumerate(v, 1):
if u != 0 and frozenset([i, j]) not in edges:
edges.add(frozenset([i, j]))
graph[i].append(j) # put graph[i].append({j: u}) if you need also to have the weight
# in this case the TSP file writing has to be modified
# for i, v in enumerate(A, 1):
# for j, u in enumerate(v, 1):
# if u != 0 and frozenset([i-1, j-1]) not in edges2:
# edges2.add(frozenset([i-1, j-1]))
# graph2[i-1].append(j-1) # put graph[i].append({j: u}) if you need also to have the weight
# in this case the TSP file writing has to be modified
return graph
def computeWeightMatrix(Adj,points):
dist = np.zeros(shape=[Adj.shape[0],Adj.shape[0]],dtype=int)
for i in range(Adj.shape[0]):
for j in range(Adj.shape[0]):
dist[i][j] = np.round(np.sqrt(np.power(points[i][0]-points[j][0],2)+np.power(points[i][1]-points[j][1],2)))
#include the tree constraints directly by weight matrix
return dist
def addConstraints(W, n_rows, n_cols, inBetweenRows):
if ( inBetweenRows ):
for i in range(1,W.shape[0]-(n_cols)):
if ( i%(n_cols+1) == 1 ):
W[i-1][i+n_cols+1] = 999
W[i+n_cols+1][i-1] = 999
elif ( i%(n_cols+1) == 0 ):
W[i-1][i+n_cols-1] = 999
W[i+n_cols-1][i-1] = 999
else:
W[i-1][i+n_cols-1] = 999
W[i-1][i+n_cols+1] = 999
W[i+n_cols-1][i-1] = 999
W[i+n_cols+1][i-1] = 999
else:
for i in range(n_rows):
for j in range(n_cols):
W[i*(2*n_cols+1) + j][(i+1) * (2*n_cols+1) +j] = 999
W[(i+1) * (2*n_cols+1) +j][i*(2*n_cols+1) + j] = 999
for i in range(n_cols):
for j in range(n_rows):
W[n_cols + j*(2*n_cols+1) + i ][n_cols +j*(2*n_cols+1) +i+1] = 999
W[n_cols +j*(2*n_cols+1) +i+1][n_cols + j*(2*n_cols+1) + i ] = 999
return W
def generateTSPFile(namefile,n_tree,distMatrix):
f = open("inputs/"+namefile,"w")
f.write( "NAME: %s" % namefile )
f.write( "\nTYPE: TSP" )
f.write( "\nCOMMENT: waypoints" )
f.write( "\nDIMENSION: %d" % (n_tree*4) )
f.write( "\nEDGE_WEIGHT_TYPE: EXPLICIT" )
f.write( "\nEDGE_WEIGHT_FORMAT: FULL_MATRIX" )
f.write( "\nEDGE_WEIGHT_SECTION\n" )
for i in range(n_tree*4):
for j in range(n_tree*4):
s = str(distMatrix[i][j])+" "
f.write(s)
s = "\n"
f.write(s)
f.write("EOF")
f.close()
def main():
params_dict = frw.getParams( 'parameters.yaml' )
#############
#store params
n_rows = params_dict['rows_number']
n_cols = params_dict['cols_number']
tree_radious = params_dict['tree_radious']
dist_row = params_dict['row_distance']
dist_col = params_dict['col_distance']
stop_time = params_dict['stop_time']
inBetweenRows = params_dict['stop_in_between']
MAX_cap_dist = params_dict['maximize_capture_distance']
obstacles_enabled = params_dict['include_trees_as_obstacles']
#############
# EVEN n_rows and n_cols brings to an ODD number of total stop points, on which the TSP algorithm would fail
if ( inBetweenRows ) and (not(n_rows%2) and not(n_cols%2)):
print("For the selected Lattice an Hamiltonian Path does not exists. Change number of stops.")
sys.exit()
# My solution
trees_pos = fc.generateTrees(n_rows,n_cols,dist_row,dist_col)
# pf.plotMapTrees(trees_pos,R)
if (inBetweenRows == 0):
capture_pts = fc.generateCapturePts(trees_pos,n_rows,n_cols, dist_row,dist_col) #ok
else:
capture_pts = fc.generateinBetweenCapturePts(n_rows,n_cols, dist_row,dist_col) #ok
# pf.plotCapturePts(capture_pts)
print(capture_pts.shape[0])
pf.plotTreesAndCaptures(trees_pos,capture_pts,tree_radious)
Adj_matrix = computeAdjMatrix(n_rows,n_cols,inBetweenRows)
W = computeWeightMatrix(Adj_matrix,capture_pts)
if ( obstacles_enabled ):
W = addConstraints(W,n_rows,n_cols,inBetweenRows)
#TODO
# W = addSteeringWeight()
Adj_list = fromMatrix2List(Adj_matrix)
frw.generateTSPFileFromAdj(inBetweenRows,capture_pts.shape[0], W*100, Adj_list) #TSP solver takes only int numbers
#TSP solver assumption: complete graph.
soluti, comp_time = computeTsp("Grid" + str(inBetweenRows) + ".tsp")
edges = fc.formatEdges(soluti.tour)
pf.plotSolutionGraph(capture_pts,edges,trees_pos,"SolGrid" + str(inBetweenRows) + str(n_rows) + "x" + str(n_cols) + ".txt")
frw.writeOutCome(stop_time,inBetweenRows, n_rows, n_cols, soluti.optimal_value/100 , soluti.tour, comp_time)
sys.exit()
#CHECK IF AN HAMILTONIAN PATH IS POSSIBLE
#FILE FORMAT:
#NAME : alb1000
#COMMENT : Hamiltonian cycle problem (Erbacci)
#TYPE : HCP
#DIMENSION : 1000
#EDGE_DATA_FORMAT : EDGE_LIST
#EDGE_DATA_SECTION
# 1000 593
# 1000 456
##### ALESSANDRO ######
centers = fc.generateCenters(n_rows,n_cols,dist_row,dist_col)
points_ref = fc.generateRefFrame(centers,R)
pf.plotCapturePts(points_ref)
if (inBetweenRows == 0): #in-between trees
thetas = np.zeros(shape=(1,n_trees))#np.pi/4#+(np.pi/2)*np.random.rand(1,n_trees)
points = fc.computePoints(thetas, points_ref, centers)
dist = distMatrix(n_trees,points,centers)
fo,sol = computeTsp(n_trees,dist,points)
edges = fc.formatEdges(sol)
pf.plotGraph(points,edges,centers,n_cols,n_rows)
pf.plotPoints(points,edges)
print(colored("inBetweenRows = 0, distance [km] : " +str(fo/molt),"cyan"))
else:
thetas45 = np.zeros(shape=(1,n_trees))+3*np.pi/4#+(np.pi/2)*np.random.rand(1,n_trees)
points45 = fc.computePoints(thetas45, points_ref, centers)
dist45 = distMatrix(n_trees,points45,centers)
fo45,sol45 = computeTsp(n_trees,dist45,points45)
edges45 = fc.formatEdges(sol45)
pf.plotGraph(points45,edges45,centers,n_cols,n_rows)
pf.plotPoints(points45,edges45)
print(colored("inBetweenRows = 45 distance [km]: " +str(fo45/molt),"green"))
# time.sleep(0.5)
# pf.plotWithoutLines(points,centers,n_cols,n_rows)
#
sys.exit()
if __name__ == '__main__':
main()
<file_sep>/pantheon_planner/functions.py
# -*- coding: utf-8 -*-
"""
Created on Sat Sep 22 19:20:08 2018
@author: Alessandro
"""
from __future__ import division
import numpy
from concorde.tsp import TSPSolver
from numpy import linalg as LA
molt = 1
'''
my functions!!
'''
def generateCapturePts(trees_pos,n_rows,n_cols,row_step,col_step):
#assumption: trees are generated row-wise from bottom to up
row_mask = createRowMask2(n_cols,col_step)
capture_pts = numpy.zeros(shape = ( n_rows * row_mask.shape[0] + n_cols, 2))
pts = numpy.zeros(shape = ( row_mask.shape[0], 2 ) )
last_pts = numpy.zeros(shape = ( n_cols, 2 ) )
for i in range(n_rows):
curr_row = i*row_step
pts[:,0] = row_mask
for j in range(n_cols + 1):
pts[j,1] = curr_row - row_step/2
for j in range(n_cols,pts.shape[0]):
pts[j,1] = curr_row
capture_pts[i*row_mask.shape[0]:(i+1)*row_mask.shape[0],:] = pts
last_row_to_draw = i*row_step
last_pts[:,0] = row_mask[0:n_cols]
for j in range(n_cols):
last_pts[j,1] = last_row_to_draw + row_step/2
capture_pts[-n_cols:,:] = last_pts
return capture_pts
def generateinBetweenCapturePts(n_rows,n_cols,row_step,col_step):
row_mask = createRowMask(n_cols,col_step)
capture_pts = numpy.zeros(shape = ( (n_rows + 1) * row_mask.shape[0], 2))
pts = numpy.zeros(shape = ( row_mask.shape[0], 2 ) )
for i in range(n_rows + 1):
curr_row = i*row_step
pts[:,0] = row_mask
pts[:,1] = curr_row - row_step/2
capture_pts[i*row_mask.shape[0]:(i+1)*row_mask.shape[0],:] = pts
return capture_pts
def createRowMask(n,step):
row_mask = numpy.zeros(shape = (n+1))
row_mask[0] = -step/2
for i in range(1,n+1,1):
row_mask[i] = row_mask[i-1] + step
return row_mask
def createRowMask2(n,step):
row_mask = numpy.zeros(shape = (2*n + 1))
row_mask[0] = 0
for i in range(1,n):
row_mask[i] = row_mask[i-1] + step
row_mask[n] = -step/2
for i in range(n+1,row_mask.shape[0]):
row_mask[i] = row_mask[i-1] + step
return row_mask
def createColMask(n,step):
col_mask = numpy.zeros(shape = (n+1))
col_mask[0] = -step/2
for i in range(1,n+1,1):
col_mask[i] = col_mask[i-1] + step
return col_mask
def readFile(name,n_nodes):
#edges = numpy.zeros(shape=(n_nodes, 2),dtype=int)
nodes = numpy.zeros(shape=(n_nodes, 1),dtype=int)
file = open(name,"r")
i=0
for line in file:
elem = line.split(" ")
for e in elem:
if e!="\n":
nodes[i,0]=int(e)
i+=1
'''for i in range(len(nodes)-1):
edges[i,:] = [nodes[i],nodes[i+1]]
edges[i+1,:] = [nodes[i+1],nodes[0]]'''
return nodes
def matlab(file):
ed = numpy.zeros(shape=(400,2),dtype=int)
file = open(file,"r")
i=0
for line in file:
ed[i][0]=line.split(" ")[0]
ed[i][1]=line.split(" ")[1]
i+=1
return ed
def getNodes(n):
nodes = []
for i in range(n):
nodes.append(str(i))
return nodes
def mapPoint(nodes,colors):
dic = {}
for n in nodes:
col = colors.get(n)
for i in range(4):
dic[(int(n)*4+i)]=col
return dic
def generateRandomCenters(num_rows, num_col):
n = num_rows*num_col
centers = numpy.zeros(shape=(n, 2))
centers[:,0]=20000 * numpy.random.rand(n)
centers[:,1]=20000 * numpy.random.rand(n)
return centers
def generateCenters(n_rows,n_col,dist_row,dist_col):
xx = 0#1*molt
yy =0#1*molt
centers = numpy.zeros(shape=(n_rows*n_col, 2))
h =0;
for i in range(0,n_rows):
for j in range(0,n_col):
centers[h,0] = xx
centers[h,1] = yy
h=h+1
xx =xx +dist_col
yy =yy +dist_row
# yy = 1*molt
xx = 0
return centers
def generateTrees(num_rows, num_cols, dist_row,dist_col):
xx = 0#1*molt
yy =0#1*molt
tree_ID = 1
centers = numpy.zeros(shape=(num_rows*num_cols, 3))
h =0;
for i in range(0,num_rows):
for j in range(0,num_cols):
centers[h,0] = xx
centers[h,1] = yy
centers[h,2] = tree_ID
h=h+1
tree_ID = tree_ID + 1
xx =xx +dist_col
yy =yy +dist_row
# yy = 1*molt
xx = 0
return centers
def generateRefFrame(centers,r):
h=0
#1.15176*molt
print(" Selected tree radious = "+ str(r))
v = numpy.matrix([[0,r],[r,0],[-r,0],[0,-r]])
points =numpy.zeros(shape =(4*centers.shape[0],2))
for i in range(centers.shape[0]):
points[h:(h+4),:]=v+centers[i,:]
h=h+4
return points
def formatEdges(solution):
edges = numpy.zeros(shape=(solution.size, 2),dtype=int)
for i in range(solution.size-1):
edges[i,:]=[solution[i],solution[i+1]]
edges[i+1,:]=[solution[i+1], solution[0]] #to close the loop
return edges
def groupByColour(dic):
dic2 ={}
for key in dic:
val = dic.get(key)
if val in dic2:
elem = dic2[val]
elem.append(key)
dic2[val]= elem
else:
elem = []
elem.append(key)
dic2[val]=elem
return dic2
def get_Trees_Edges(neighbors,n_trees):
n_edges_i = []
n_edges_j = []
h=0
for key in neighbors:
elem = neighbors.get(key)
for i in range(len(elem)):
n_edges_i.append(int(key))
n_edges_j.append(int(elem[i]))
h+=1
return n_edges_i,n_edges_j
def rotMatrix(x,y,theta,cx,cy):
p = numpy.zeros(shape=(1, 2))
new_x = x-cx;
new_y = y-cy;
p[0][0]= new_x*numpy.cos(theta)-new_y*numpy.sin(theta)+ cx
p[0][1]= new_x*numpy.sin(theta)+new_y*numpy.cos(theta)+ cy
return p
| 7045b5140ea9001f8c6779ea573041fe3e890b9d | [
"Markdown",
"Python"
] | 4 | Python | project-pantheon/pantheon_glob_planner | c0d50a53b36c4678192ec75ad7a4cd68c570daef | 3d1b39b453d51e654acece990d3d96c6b5fa1c28 | |
refs/heads/master | <file_sep>package com.xinnuo.apple.nongda.TeacherActivity.CourseAttendance;
/**
* 查询所有教师
* */
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.TeacherActivity.Substitute.TeacherSubstituteAddActivity;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class AllTeachersActivity extends BaseActivity {
private OkHttpClient client;
private ListView listView;
private String teacherId;
private JSONArray dataArr;
private int positions;
private String name;
private String state;
private String coordinateId;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_all_teachers);
listView = (ListView) findViewById(R.id.all_teacher);
Intent intent = getIntent();
coordinateId = intent.getStringExtra("coordinateId");
teacherId = intent.getStringExtra("teacherId");
state = intent.getStringExtra("state");
initOkHttp();
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
try {
Intent intent=new Intent(AllTeachersActivity.this,TeacherSubstituteAddActivity.class);
JSONObject js = dataArr.getJSONObject(position);
positions = position;
// 设置显示信息
final AlertDialog.Builder builder = new AlertDialog.Builder(AllTeachersActivity.this);
builder.setMessage("确定要提交?").
// 设置确定按钮
setPositiveButton("是",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which)
{
//点击相应的班级进行跳转
try {
if (state.equals("1"))
{
Intent intent=new Intent(AllTeachersActivity.this,SubstituteCourseActivity.class);
JSONObject js = dataArr.getJSONObject(positions);
//传值 班级id 所教班级id
intent.putExtra("substituteId",js.getString("substituteId"));
intent.putExtra("teacherId",teacherId);
intent.putExtra("teacherId1",js.getString("teacherId"));
intent.putExtra("teacherName",js.getString("teacherName"));
intent.putExtra("itemId",js.getString("itemId"));
Log.d("name = ",name);
startActivity(intent);
}else
{
Intent intent=new Intent(AllTeachersActivity.this,CombinedLessonActivity.class);
JSONObject js = dataArr.getJSONObject(positions);
//传值 班级id 所教班级id
intent.putExtra("substituteId",js.getString("substituteId"));
intent.putExtra("teacherId",teacherId);
intent.putExtra("teacherId1",js.getString("teacherId"));
intent.putExtra("teacherName",js.getString("teacherName"));
intent.putExtra("itemId",js.getString("itemId"));
Log.d("name = ",name);
startActivity(intent);
}
finish();
} catch (JSONException e) {
e.printStackTrace();
}
}
}).
// 设置取消按钮
setNegativeButton("否",
new DialogInterface.OnClickListener()
{
public void onClick(DialogInterface dialog,
int which)
{
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
} catch (JSONException e) {
e.printStackTrace();
}
}
});
}
@Override
protected void onStart() {
super.onStart();
requestWithUserId();
}
/**
* 请求方法
* */
private void requestWithUserId(){
//菊花的开始方法
mLoading.show();
RequestBody requestBodyPost = new FormBody.Builder()
.add("subTeacherId",teacherId)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.TeacherSubstituteTeachersQuery)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
mLoading.dismiss();
e.printStackTrace();
}
}
});
}
});
}
/**
* 解析数据方法
* */
//json解析方法
private void jsonParseWithJsonStr (String jsonStr) throws JSONException {
JSONArray jsArr = new JSONArray(jsonStr);
dataArr = jsArr;
List<String> data = new ArrayList<String>();
ArrayList<HashMap<String, Object>> listItem = new ArrayList<HashMap<String, Object>>();/*在数组中存放数据*/
HashMap<String, Object> map2 = new HashMap<String, Object>();
for (int i = 0; i < jsArr.length(); i++) {
JSONObject js = jsArr.getJSONObject(i);
name = js.optString("teacherName");
HashMap<String, Object> map = new HashMap<String, Object>();
map.put("name", name);
listItem.add(map);
}
if (jsArr.length() == 0)
{
midToast("无数据",3);
}
SimpleAdapter qrAdapter = new SimpleAdapter(this,listItem, R.layout.item_all_teacher,
new String[]{"name"},
new int[]{R.id.all_teacher1});
listView.setAdapter(qrAdapter);
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda.studentActivity.AccountSettings;
/**
* 账号设置界面 里面包含5个功能
* */
import android.app.AlertDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import android.widget.TextView;
import com.xinnuo.apple.nongda.Binding.StudentBindingActivity;
import com.xinnuo.apple.nongda.MainActivity;
import com.xinnuo.apple.nongda.R;
public class StuAccountSettingsActivity extends AppCompatActivity {
//定义控件
private TextView stu_account_setting1; //绑定手机号
private TextView stu_account_setting2; //修改密码
private TextView stu_account_setting3; //意见反馈
private TextView stu_account_setting4; //关于我们
private TextView stu_sign_out; //退出登录
private String studentNo;
private String id;
private String password;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_stu_account_settings);
Intent intent = getIntent();
id = intent.getStringExtra("id");
studentNo = intent.getStringExtra("studentNo");
Log.d("stuNo =",studentNo);
password = intent.getStringExtra("password");
binding();
clickJump();
}
//绑定控件
private void binding()
{
stu_account_setting1 = (TextView) findViewById(R.id.stu_account_setting1);
stu_account_setting2 = (TextView) findViewById(R.id.stu_account_setting2);
stu_account_setting3 = (TextView) findViewById(R.id.stu_account_setting3);
stu_account_setting4 = (TextView) findViewById(R.id.stu_account_setting4);
stu_sign_out = (TextView) findViewById(R.id.sign_out);
}
//点击相应控件进行相应的跳转
private void clickJump()
{
//绑定手机号
stu_account_setting1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(StuAccountSettingsActivity.this,StudentBindingActivity.class);
intent.putExtra("id",id);
String state = "2";
intent.putExtra("studentNo",studentNo);
intent.putExtra("password",<PASSWORD>);
intent.putExtra("state",state);
startActivity(intent);
}
});
//修改密码
stu_account_setting2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(StuAccountSettingsActivity.this,StuModifyPasswordActivity.class);
intent.putExtra("id",id);
intent.putExtra("studentNo",studentNo);
intent.putExtra("password",<PASSWORD>);
startActivity(intent);
}
});
//意见反馈
stu_account_setting3.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
}
});
//关于我们
stu_account_setting4.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
}
});
//退出登录
stu_sign_out.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
loginOut();
}
});
}
//退出方法 点击注销时给出提示信息
private void loginOut(){
// 设置显示信息
final AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage("确定要退出?").
// 设置确定按钮
setPositiveButton("是",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which) {
// 设置TextView文本
//点击是的时候去进行提交
SharedPreferences sp = getSharedPreferences("userinfo",
Context.MODE_PRIVATE);
SharedPreferences.Editor editor = sp.edit();
editor.putBoolean("pswd_checkBox", false);
editor.putString("msgDate", null);
editor.commit();
//进行页面跳转,跳回登录界面
Intent intent = new Intent(StuAccountSettingsActivity.this, MainActivity.class);
startActivity(intent);
finish();
}
}).
// 取消跳转
setNegativeButton("否",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
}
}
<file_sep>package com.xinnuo.apple.nongda.entity;
/**
* 教师基类
*/
public class TeacherModel {
/**
* birthday :
* phone : 18904509588
* sex : 1
* status : 3
* jurisdiction : 3
* itemId : 1001
* collegeName :
* password : 1
* intro : 3
* id : 27
* cardNo : 12345678
* picture : t01b79970053ab4ab15.jpg
* nationName : 汉族
* name : 杨俊伟
* loginSta : success
*/
private String birthday;
private String phone;
private int sex;
private int status;
private String jurisdiction;
private String itemId;
private String collegeName;
private String password;
private String intro;
private String id;
private String cardNo;
private String picture;
private String nationName;
private String name;
private String loginSta;
public String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
this.birthday = birthday;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public int getSex() {
return sex;
}
public void setSex(int sex) {
this.sex = sex;
}
public int getStatus() {
return status;
}
public void setStatus(int status) {
this.status = status;
}
public String getJurisdiction() {
return jurisdiction;
}
public void setJurisdiction(String jurisdiction) {
this.jurisdiction = jurisdiction;
}
public String getItemId() {
return itemId;
}
public void setItemId(String itemId) {
this.itemId = itemId;
}
public String getCollegeName() {
return collegeName;
}
public void setCollegeName(String collegeName) {
this.collegeName = collegeName;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getIntro() {
return intro;
}
public void setIntro(String intro) {
this.intro = intro;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getCardNo() {
return cardNo;
}
public void setCardNo(String cardNo) {
this.cardNo = cardNo;
}
public String getPicture() {
return picture;
}
public void setPicture(String picture) {
this.picture = picture;
}
public String getNationName() {
return nationName;
}
public void setNationName(String nationName) {
this.nationName = nationName;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getLoginSta() {
return loginSta;
}
public void setLoginSta(String loginSta) {
this.loginSta = loginSta;
}
}
<file_sep>package com.xinnuo.apple.nongda.TeacherActivity.Resultquery;
/**
* 展示学生列表
* */
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class StudentDetailedQueryActivity extends BaseActivity {
private OkHttpClient client;
private ListView listView;
private String studentId;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_student_detailed_query);
initOkHttp();
listView = (ListView) findViewById(R.id.list_studentDetaileds) ;
}
protected void onStart()
{
super.onStart();
//取出上个界面传过来的id
Intent intent = getIntent();
studentId = intent.getStringExtra("studentId");
requestWithUserId(studentId);
}
//请求方法
private void requestWithUserId(String id){
mLoading.show();
Log.d("StudentList ==== ", id);
RequestBody requestBodyPost = new FormBody.Builder()
.add("studentId",id) //id
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.StudentDetailedScore) //请求地址
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收后台返回值方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
e.printStackTrace();
}
}
});
}
});
}
//json解析
/*
* 测试数据
* "result": "51.60",
"sex": "2",
"weight": "54.3",
"gradeId": "4",
"vital_capacity_mark": "0.0",
"jump_mark": "100.0",
"name": "董萍",
"studentNo": "12011101",
"kiol_awarded_marks": "0",
"vital_capacity_evaluate": "不及格",
"stature": "166.3",
"kilo_evaluate": "0",
"jump_evaluate": "优秀",
"bend_evaluate": "及格",
"kilo_capacity": "0",
"lie_capacity": "0",
"health": "不及格",
"kilo_mark": "0",
"jump": "211",
"lie_awarded_marks": "0",
"vital_capacity": "641",
"Bmi_mark": "100.0",
"bendl_capacity": "11.0",
"fifty_mark": "100.0",
"lie_mark": "0",
"bmi_evaluate": "100.0",
"fifty_evaluate": "优秀",
"lie_evaluate": "0",
"bend_mark": "66.0",
"fifty_capacity": "6.53"
* */
private void jsonParseWithJsonStr (String jsonStr) throws JSONException {
ArrayList<HashMap<String, Object>> listItem = new ArrayList<HashMap<String, Object>>();/*在数组中存放数据*/
JSONObject js = new JSONObject(jsonStr);
HashMap<String, Object> map = new HashMap<String, Object>();
map.put("Item1",js.getString("name"));
map.put("Item2", js.getString("studentNo"));
String sex;
if (js.getString("sex").equals("1")) {
sex= "男";
}else{
sex="女";
}
map.put("Item3", sex);
String gradeId;
if (js.getString("gradeId").equals("1"))
{
gradeId = "一年级";
}else if (js.getString("gradeId").equals("2"))
{
gradeId = "二年级";
}else if (js.getString("gradeId").equals("3"))
{
gradeId = "三年级";
}else
{
gradeId = "四年级";
}
map.put("Item4", gradeId);
//成绩
map.put("Item6", js.getString("stature"));
map.put("Item7", js.getString("weight"));
map.put("Item8", js.getString("vital_capacity"));
map.put("Item9", js.getString("jump"));
map.put("Item10", js.getString("lie_capacity"));
map.put("Item11", js.getString("bendl_capacity"));
map.put("Item12", js.getString("fifty_capacity"));
map.put("Item13", js.getString("kilo_capacity"));
map.put("Item14", js.getString("lie_awarded_marks"));
map.put("Item15", js.getString("kiol_awarded_marks"));
//得分
map.put("Item16", js.getString("Bmi_mark"));
map.put("Item17", js.getString("Bmi_mark"));
map.put("Item18", js.getString("vital_capacity_mark"));
map.put("Item19", js.getString("jump_mark"));
map.put("Item20", js.getString("lie_mark"));
map.put("Item21", js.getString("bend_mark"));
map.put("Item22", js.getString("fifty_mark"));
map.put("Item23", js.getString("kilo_mark"));
map.put("Item24", "0");
map.put("Item25", "0");
map.put("Item36", js.getString("result"));
//评价
map.put("Item26", js.getString("bmi_evaluate"));
map.put("Item27", js.getString("bmi_evaluate"));
map.put("Item28", js.getString("vital_capacity_evaluate"));
map.put("Item29", js.getString("jump_evaluate"));
map.put("Item30", js.getString("lie_evaluate"));
map.put("Item31", js.getString("bend_evaluate"));
map.put("Item32", js.getString("fifty_evaluate"));
map.put("Item33", js.getString("kilo_evaluate"));
map.put("Item34", " ");
map.put("Item35", " ");
map.put("Item37", js.getString("health"));
listItem.add(map);
if (js.length() == 0)
{
midToast("无数据",3);
}
SimpleAdapter qrAdapter;
if (js.getString("sex").equals("1")) {
qrAdapter = new SimpleAdapter(this, listItem, R.layout.item_student_detailed_boy_query,
new String[]{"Item1", "Item2", "Item3", "Item4", "Item6", "Item7", "Item8", "Item9", "Item10", "Item11", "Item12", "Item13", "Item14", "Item15", "Item16", "Item17", "Item18", "Item19", "Item20",
"Item21", "Item22", "Item23", "Item24", "Item25", "Item26", "Item27", "Item28", "Item29", "Item30", "Item31", "Item32", "Item33", "Item34", "Item35", "Item36", "Item37"},
new int[]{R.id.detailed56, R.id.detailed58, R.id.detailed52, R.id.detailed54, R.id.detailed8, R.id.detailed13, R.id.detailed18, R.id.detailed23, R.id.detailed28, R.id.detailed33
, R.id.detailed38, R.id.detailed43, R.id.detailed48, R.id.detailed61, R.id.detailed9, R.id.detailed14, R.id.detailed19, R.id.detailed24, R.id.detailed29, R.id.detailed34,
R.id.detailed39, R.id.detailed44, R.id.detailed49, R.id.detailed62, R.id.detailed10, R.id.detailed15, R.id.detailed20, R.id.detailed25, R.id.detailed30, R.id.detailed35,
R.id.detailed40, R.id.detailed45, R.id.detailed50, R.id.detailed63, R.id.detailed67, R.id.detailed68});
}else {
qrAdapter = new SimpleAdapter(this, listItem, R.layout.item_student_detailed_girl_query,
new String[]{"Item1", "Item2", "Item3", "Item4", "Item6", "Item7", "Item8", "Item9", "Item10", "Item11", "Item12", "Item13", "Item14", "Item15", "Item16", "Item17", "Item18", "Item19", "Item20",
"Item21", "Item22", "Item23", "Item24", "Item25", "Item26", "Item27", "Item28", "Item29", "Item30", "Item31", "Item32", "Item33", "Item34", "Item35", "Item36", "Item37"},
new int[]{R.id.detailed56, R.id.detailed58, R.id.detailed52, R.id.detailed54, R.id.detailed8, R.id.detailed13, R.id.detailed18, R.id.detailed23, R.id.detailed28, R.id.detailed33
, R.id.detailed38, R.id.detailed43, R.id.detailed48, R.id.detailed61, R.id.detailed9, R.id.detailed14, R.id.detailed19, R.id.detailed24, R.id.detailed29, R.id.detailed34,
R.id.detailed39, R.id.detailed44, R.id.detailed49, R.id.detailed62, R.id.detailed10, R.id.detailed15, R.id.detailed20, R.id.detailed25, R.id.detailed30, R.id.detailed35,
R.id.detailed40, R.id.detailed45, R.id.detailed50, R.id.detailed63, R.id.detailed67, R.id.detailed68});
}
listView.setAdapter(qrAdapter);
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda.TeacherActivity.CourseAttendance;
/**
* 考勤列表
* */
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class TeacherAttendanceListActivity extends BaseActivity {
private OkHttpClient client;
private String sportclassId;
private ListView listView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_teacher_attendance_list);
listView = (ListView) findViewById(R.id.teacher_attendance_list);
initOkHttp();
Intent intent = getIntent();
sportclassId = intent.getStringExtra("sportclassId");
requestWithUserId();
}
/**
* 请求方法
* */
private void requestWithUserId(){
//菊花的开始方法
mLoading.show();
RequestBody requestBodyPost = new FormBody.Builder()
.add("sportclassId",sportclassId)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.TeacherAttendanceList)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
mLoading.dismiss();
e.printStackTrace();
}
}
});
}
});
}
/**
* 解析数据
* 测试数据
* "sex": "2",
"name": "石健美",
"sportClass": "乒乓球",
"type": "no",
"sutdentNo": "11042220"
* */
private void jsonParseWithJsonStr (String jsonStr) throws JSONException {
JSONArray jsArr = new JSONArray(jsonStr);
List<String> data = new ArrayList<String>();
ArrayList<HashMap<String, Object>> listItem = new ArrayList<HashMap<String, Object>>();/*在数组中存放数据*/
for (int i = 0; i < jsArr.length(); i++) {
JSONObject js = jsArr.getJSONObject(i);
String count = i+1+"";
String sutdentNo = js.getString("sutdentNo");
String name = js.getString("name");
String sex;
if (js.getString("sex").equals("1"))
{
sex = "男";
}else
{
sex = "女";
}
String sportClass = js.getString("sportClass");
String type ;
if (js.getString("type").equals("yes"))
{
type = js.getString("date");
}else
{
type = "未签到";
}
HashMap<String, Object> map = new HashMap<String, Object>();
map.put("count",count);
map.put("sutdentNo",sutdentNo);
map.put("name",name);
map.put("sex",sex);
map.put("sportClass",sportClass);
map.put("type",type);
listItem.add(map);
}
if (jsArr.length() == 0)
{
midToast("无数据",3);
}
SimpleAdapter qrAdapter = new SimpleAdapter(this,listItem, R.layout.item_teacher_attendance_list,
new String[]{"count","sutdentNo","name","sex","sportClass","type"},
new int[]{R.id.tea_att_list_number, R.id.tea_att_list_stunumber, R.id.tea_att_list_name, R.id.tea_att_list_sex, R.id.tea_att_list_class, R.id.tea_att_list_time,});
listView.setAdapter(qrAdapter);
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda.TeacherActivity.WorkPlan;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import android.widget.TextView;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class TeacherWorkPlan extends BaseActivity {
private ListView listView;
private OkHttpClient client;
private String teacherId;
private TextView newlyAdded;
private JSONArray dataArr;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
initOkHttp();
setContentView(R.layout.activity_teacher_work_plan);
listView = (ListView) findViewById(R.id.list_work_plan);
newlyAdded = (TextView) findViewById(R.id.newly_added);
final Intent intent = getIntent();
teacherId = intent.getStringExtra("teacherId");
requestWithUserId(teacherId);
newlyAdded.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent1 = new Intent(TeacherWorkPlan.this,TeacherAddWorkPlan.class);
intent1.putExtra("teacherId",teacherId);
startActivity(intent1);
}
});
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intent1 = new Intent(TeacherWorkPlan.this,TeacherUpdateWorkPlan.class);
try {
JSONObject work = dataArr.getJSONObject(position);
String ids = work.getString("id");
String title = work.getString("title");
String createDate = work.getString("createDate");
String beginDate = work.getString("beginDate");
String endDate = work.getString("endDate");
String workContent = work.getString("workContent");
String state = work.getString("state");
String summary = work.getString("summary");
Log.d("****",state+workContent+endDate+beginDate);
intent1.putExtra("id",ids);
intent1.putExtra("title",title);
intent1.putExtra("createDate",createDate);
intent1.putExtra("beginDate",beginDate);
intent1.putExtra("endDate",endDate);
intent1.putExtra("workContent",workContent);
intent1.putExtra("state",state);
intent1.putExtra("summary",summary);
intent1.putExtra("teacherId",teacherId);
startActivity(intent1);
} catch (JSONException e) {
e.printStackTrace();
}
}
});
}
/**
* 请求方法
* */
private void requestWithUserId(String teacherId){
//菊花的开始方法
mLoading.show();
RequestBody requestBodyPost = new FormBody.Builder()
.add("teacherId",teacherId)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.WorkPlan)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
mLoading.dismiss();
e.printStackTrace();
}
}
});
}
});
}
/**
* 解析数据方法
* */
private void jsonParseWithJsonStr (String jsonStr) throws JSONException {
JSONArray jsArr = new JSONArray(jsonStr);
dataArr = jsArr;
List<String> data = new ArrayList<String>();
ArrayList<HashMap<String, Object>> listItem = new ArrayList<HashMap<String, Object>>();/*在数组中存放数据*/
for (int i = 0; i < jsArr.length(); i++)
{
JSONObject js = jsArr.getJSONObject(i);
String title = js.optString("title");
String createDate = js.getString("createDate");
String beginDate = js.getString("beginDate");
String endDate = js.getString("endDate");
String workContent = js.getString("workContent");
String state = js.getString("state");
String summary = js.getString("summary");
HashMap<String, Object> map = new HashMap<String, Object>();
map.put("title",title);
map.put("createDate",createDate);
map.put("beginDate",beginDate);
map.put("endDate",endDate);
map.put("workContent",workContent);
map.put("state",state);
map.put("summary",summary);
listItem.add(map);
}
if (jsArr.length() == 0)
{
midToast("无数据",3);
}
SimpleAdapter qrAdapter = new SimpleAdapter(this,listItem, R.layout.item_teacher_work_plan,
new String[]{"title","createDate","beginDate","endDate","workContent","state","summary"},
new int[]{R.id.work_title, R.id.work_createdate, R.id.work_startdate, R.id.work_enddate, R.id.work_plan, R.id.work_yesno, R.id.work_summary});
listView.setAdapter(qrAdapter);
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda.singin;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.util.Log;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.entity.StudentModel;
import com.xinnuo.apple.nongda.Binding.StudentBindingActivity;
import com.xinnuo.apple.nongda.studentActivity.StudentActivity;
import org.json.JSONException;
import org.json.JSONObject;
/**
* 学生登录方法
* stu_Login 跳转方法 setSutdentInfoWith 学生对象赋值方法 stu_Binding 跳转学生绑定方法
*/
public class Student_Singin extends BaseActivity {
/**
* 学生跳转方法:登陆成功跳转到学生操作界面
* 传的值 studentId:学生id studentNo:学号 studentClassId:班级id studentName:学生姓名及班级名称:
* */
public Intent stu_Login(Activity activity,JSONObject jsObj , StudentModel studentInfo)
{
Intent intent = null;
try {
studentInfo = setSutdentInfoWith(jsObj);
//跳转界面
intent = new Intent(activity, StudentActivity.class);
Log.d("student id = ", studentInfo.getId());
//传值 学生id 学生学号 班级id 学生姓名 班级名称
intent.putExtra("studentId", studentInfo.getId());
intent.putExtra("studentNo", studentInfo.getStudentNo());
intent.putExtra("studentClassId", studentInfo.getClassId());
intent.putExtra("studentName",studentInfo.getName());
intent.putExtra("className",studentInfo.getClassName());
intent.putExtra("password",studentInfo.getPassword());
Integer gId = studentInfo.getGradeId();
intent.putExtra("gradeId",gId.toString());
// Log.d("~~~~~mainclassId = ", studentInfo.getClassId()+"\n"+studentInfo.getStudentNo()+"\n"+studentInfo.getName()+"\n"+studentInfo.getClassName());
} catch (JSONException e) {
e.printStackTrace();
}
return intent;
}
/**
* 为学生对象赋值方法
* */
public StudentModel setSutdentInfoWith(JSONObject jsObj) throws JSONException
{
//学生model
StudentModel student = new StudentModel();
student.setCardId(jsObj.getString("cardId"));
student.setBirthday(jsObj.getString("birthday"));
student.setPhone(jsObj.getString("phone"));
student.setStudentNo(jsObj.getString("studentNo"));
student.setSex(jsObj.getString("sex"));
student.setGradeId(jsObj.getInt("gradeId"));
student.setStatus(jsObj.getInt("status"));
student.setClassId(jsObj.getString("classId"));
student.setElectiveInformation(jsObj.getString("electiveInformation"));
student.setPassword(<PASSWORD>("<PASSWORD>"));
student.setCollegeName(jsObj.getString("collegeName"));
student.setNationId(jsObj.getString("nationId"));
student.setId(jsObj.getString("id"));
student.setStuSource(jsObj.getString("stuSource"));
student.setHomeAddress(jsObj.getString("homeAddress"));
student.setName(jsObj.getString("name"));
student.setClassName(jsObj.getString("className"));
student.setLoginSta(jsObj.getString("loginSta"));
student.setReelect(jsObj.getInt("reelect"));
student.setIdNumber(jsObj.getString("idNumber"));
return student;
}
/**
*学生绑定方法 跳转到学生绑定界面
* 传的值 stuNo 学号
* */
public void stu_Binding(final String stuNo, final Activity activity ,AlertDialog.Builder builder) throws Exception
{
//final String userNo = userIdEditText.getText().toString().trim();
// 设置显示信息
builder.setMessage("身份未绑定,是否绑定?").
// 设置确定按钮
setPositiveButton("是",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which) {
//进行页面跳转 跳到绑定界面需要输入身份证号和手机号进行绑定
Intent intent = new Intent(activity, StudentBindingActivity.class);
//传值学生id
intent.putExtra("stuNo", stuNo);
}
}).
// 设置取消按钮
setNegativeButton("否",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
}
}
<file_sep>package com.xinnuo.apple.nongda.Admin.Meeting;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.CheckBox;
import android.widget.CompoundButton;
import android.widget.CompoundButton.OnCheckedChangeListener;
import android.widget.TextView;
import com.xinnuo.apple.nongda.R;
import java.util.List;
public class Myadapter extends BaseAdapter {
private List<String> arraylist;
private List<String> arraylist1;
private List<String> arraylist2;
private List<String> arraylist3;
private Context context;
private boolean[] array;
public Myadapter(Context context, List<String> arraylist,List<String> arraylist1,List<String> arraylist2,List<String> arraylist3, boolean[] array ){
this.context = context;
this.arraylist = arraylist;
this.arraylist1 = arraylist1;
this.arraylist2 = arraylist2;
this.arraylist3 = arraylist3;
this.array = array;
}
@Override
public View getView(final int position, View convertView,
ViewGroup parent) {
// TODO Auto-generated method stub
ViewHolder vh = new ViewHolder();
if (convertView == null) {
convertView = LayoutInflater.from(context).inflate(R.layout.item_query_all_teacher, null);
vh.tv = (TextView) convertView.findViewById(R.id.admin_number);
vh.tv1 = (TextView) convertView.findViewById(R.id.admin_name);
vh.tv2 = (TextView) convertView.findViewById(R.id.admin_subject);
vh.tv3 = (TextView) convertView.findViewById(R.id.admin_phone);
vh.cb = (CheckBox) convertView.findViewById(R.id.checkBox);
convertView.setTag(vh);
}else{
vh = (ViewHolder) convertView.getTag();
}
if(AdminQueryAllteacherActivity.visiblecheck){
vh.cb.setVisibility(View.VISIBLE);
}else{
vh.cb.setVisibility(View.GONE);
}
vh.cb.setOnCheckedChangeListener(new OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView,
boolean isChecked) {
// TODO Auto-generated method stub
array[position] = isChecked;
}
});
vh.tv.setText(arraylist.get(position));
vh.tv1.setText(arraylist1.get(position));
vh.tv2.setText(arraylist2.get(position));
vh.tv3.setText(arraylist3.get(position));
vh.cb.setChecked(array[position]);
return convertView;
}
class ViewHolder {
public TextView tv;
public TextView tv1;
public TextView tv2;
public TextView tv3;
public CheckBox cb;
}
@Override
public int getCount() {
// TODO Auto-generated method stub
return arraylist.size();
}
@Override
public Object getItem(int position) {
// TODO Auto-generated method stub
return arraylist.get(position);
}
@Override
public long getItemId(int position) {
// TODO Auto-generated method stub
return position;
}
}
<file_sep>package com.xinnuo.apple.nongda.Admin.Meeting;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.TextView;
import com.amap.api.location.AMapLocation;
import com.amap.api.location.AMapLocationClient;
import com.amap.api.location.AMapLocationClientOption;
import com.amap.api.location.AMapLocationListener;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.Utils;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class MeetingInfoDetailActivity extends BaseActivity {
private OkHttpClient client;
private TextView leave;
private TextView sign;
private TextView conferencelist;
private TextView relTime;
private TextView meetType;
private TextView teacherinfoName;
private TextView theme;
private TextView meetingTime;
private TextView place;
private TextView introduction;
private String id;
private String teacherId;
private String relTime1;
private String meetType1;
private String teacherinfoName1;
private String theme1;
private String meetingTime1;
private String place1;
private String introduction1;
private static double signLongitude;
private static double signLatitude;
private AMapLocationClient locationClient = null;
private AMapLocationClientOption locationOption = new AMapLocationClientOption();
private static final double ALERT_DISTANCE = 50;
private static double distance;
private static double Longitude;
private static double Latitude;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_meeting_info_detail);
Binding();
initOkHttp();
final Intent intent = getIntent();
teacherId = intent.getStringExtra("teacherId");
relTime.setText(intent.getStringExtra("relTime"));
relTime1 = intent.getStringExtra("relTime");
meetType1 = intent.getStringExtra("meetType");
teacherinfoName1 = intent.getStringExtra("teacherinfoName");
theme1 = intent.getStringExtra("theme");
meetingTime1 = intent.getStringExtra("meetingTime");
place1 = intent.getStringExtra("place");
introduction1 = intent.getStringExtra("introduction");
id = intent.getStringExtra("id");
String meetTypes;
if (intent.getStringExtra("meetType").equals("0"))
{
meetTypes = "签到未开始";
}else if (intent.getStringExtra("meetType").equals("1"))
{
meetTypes = "签到进行中,请准备签到";
}else
{
meetTypes = "签到已结束";
}
meetType.setText(meetTypes);
teacherinfoName.setText(intent.getStringExtra("teacherinfoName"));
theme.setText(intent.getStringExtra("theme"));
meetingTime.setText(intent.getStringExtra("meetingTime"));
place.setText(intent.getStringExtra("place"));
introduction.setText(intent.getStringExtra("introduction"));
id = intent.getStringExtra("id");
Log.d("id======",id);
conferencelist.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
initLocation();
startLocation();
}
});
leave.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 设置显示信息
final AlertDialog.Builder builder = new AlertDialog.Builder(MeetingInfoDetailActivity.this);
builder.setMessage("确定要删除会议?").
// 设置确定按钮
setPositiveButton("是",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which)
{
// 设置TextView文本
//点击是的时候去进行提交
scanCodeSign(id);
}
}).
// 设置取消按钮
setNegativeButton("否",
new DialogInterface.OnClickListener()
{
public void onClick(DialogInterface dialog,
int which)
{
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
}
});
sign.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent1 = new Intent(MeetingInfoDetailActivity.this,AdminAttendanceListActivity.class);
intent1.putExtra("id",id);
startActivity(intent1);
}
});
}
//绑定控件方法
private void Binding()
{
leave = (TextView) findViewById(R.id.meet_leave);
sign= (TextView) findViewById(R.id.meet_sign);
conferencelist = (TextView) findViewById(R.id.meet_conferencelist);
relTime = (TextView) findViewById(R.id.admin_meeting_info1);
meetType = (TextView) findViewById(R.id.admin_meeting_info2);
teacherinfoName = (TextView) findViewById(R.id.admin_meeting_info3);
theme = (TextView) findViewById(R.id.admin_meeting_info4);
meetingTime = (TextView) findViewById(R.id.admin_meeting_info5);
place = (TextView) findViewById(R.id.admin_meeting_info6);
introduction = (TextView) findViewById(R.id.admin_meeting_info7);
}
private void initLocation(){
//初始化client
locationClient = new AMapLocationClient(this.getApplicationContext());
//设置定位参数
locationClient.setLocationOption(getDefaultOption());
// 设置定位监听
locationClient.setLocationListener(locationListener);
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
/**
* 开始定位
*
* @since 2.8.0
* @author hongming.wang
*
*/
private void startLocation(){
// 设置定位参数
locationClient.setLocationOption(locationOption);
// 启动定位
locationClient.startLocation();
}
/**
* 默认的定位参数
* @since 2.8.0
* @author hongming.wang
*
*/
private AMapLocationClientOption getDefaultOption(){
AMapLocationClientOption mOption = new AMapLocationClientOption();
mOption.setLocationMode(AMapLocationClientOption.AMapLocationMode.Hight_Accuracy);//可选,设置定位模式,可选的模式有高精度、仅设备、仅网络。默认为高精度模式
mOption.setGpsFirst(false);//可选,设置是否gps优先,只在高精度模式下有效。默认关闭
mOption.setHttpTimeOut(30000);//可选,设置网络请求超时时间。默认为30秒。在仅设备模式下无效
mOption.setInterval(2000);//可选,设置定位间隔。默认为2秒
mOption.setNeedAddress(true);//可选,设置是否返回逆地理地址信息。默认是ture
mOption.setOnceLocation(true);//可选,设置是否单次定位。默认是false
mOption.setOnceLocationLatest(false);//可选,设置是否等待wifi刷新,默认为false.如果设置为true,会自动变为单次定位,持续定位时不要使用
AMapLocationClientOption.setLocationProtocol(AMapLocationClientOption.AMapLocationProtocol.HTTP);//可选, 设置网络请求的协议。可选HTTP或者HTTPS。默认为HTTP
return mOption;
}
/**
* 定位监听
*/
AMapLocationListener locationListener = new AMapLocationListener() {
@Override
public void onLocationChanged(AMapLocation loc) {
if (null != loc) {
//解析定位结果
String result = Utils.getLocationStr(loc);
/**
* sb.append("经 度 : " + location.getLongitude() + "\n");
sb.append("纬 度 : " + location.getLatitude() + "\n");
*/
if (Latitude == 0.0 && Longitude == 0.0) {
Longitude = loc.getLongitude();
Latitude = loc.getLatitude();
final String coutent = "教师已上传定位坐标,可以定位签到了";
startPositioning();
}
String ret = ""+Longitude + Latitude;
String jStr = Longitude+",";
String wStr = Latitude+"";
Log.d("定位结果:long = ",Longitude+"lat = "+Latitude);
// statuTV.setText(jStr+wStr);
// test3TV.setText("定位结果:long = "+Longitude+"lat = "+Latitude);
} else {
//statuTV.setText("定位失败,loc is null");
}
}
};
/**
* 根据两点间经纬度坐标(double值),计算两点间距离,
*
* @param lat1
* @param lng1
* @param lat2
* @param lng2
* @return 距离:单位为米
*/
public static double DistanceOfTwoPoints(double lat1,double lng1,
double lat2,double lng2) {
double radLat1 = rad(lat1);
double radLat2 = rad(lat2);
double a = radLat1 - radLat2;
double b = rad(lng1) - rad(lng2);
double s = 2 * Math.asin(Math.sqrt(Math.pow(Math.sin(a / 2), 2)
+ Math.cos(radLat1) * Math.cos(radLat2)
* Math.pow(Math.sin(b / 2), 2)));
s = s * 6378137.0;
s = Math.round(s * 10000) / 10000;
return s;
}
/**
* 停止定位
*
* @since 2.8.0
* @author hongming.wang
*
*/
private void stopLocation(){
// 停止定位
locationClient.stopLocation();
}
@Override
protected void onDestroy() {
super.onDestroy();
destroyLocation();
}
/**
* 销毁定位
*
* @since 2.8.0
* @author hongming.wang
*
*/
private void destroyLocation(){
if (null != locationClient) {
/**
* 如果AMapLocationClient是在当前Activity实例化的,
* 在Activity的onDestroy中一定要执行AMapLocationClient的onDestroy
*/
locationClient.onDestroy();
locationClient = null;
locationOption = null;
}
}
private static double rad(double d) {
return d * Math.PI / 180.0;
}
//存入管理员坐标
private void startPositioning(){
RequestBody requestBodyPost = new FormBody.Builder()
.add("meetingId",id)
.add("longitude",Longitude+"")
.add("latitude",Latitude+"")
.add("meetType","1")
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.MeetingOpenSignIn)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
mLoading.dismiss();
try {
JSONObject jsObj = new JSONObject(retStr);
if (jsObj.getString("melodyClass").equals("yes")){
AlertDialog.Builder builder = new AlertDialog.Builder(MeetingInfoDetailActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("坐标已上传,可以开始签到了!") ;
String ret = ""+Longitude + Latitude;
Log.d("ret = ",ret);
builder.setPositiveButton("确定" , null );
builder.show();
}else if (jsObj.getString("melodyClass").equals("no")){
AlertDialog.Builder builder = new AlertDialog.Builder(MeetingInfoDetailActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("坐标上传失败!") ;
String ret = ""+Longitude + Latitude;
Log.d("ret = ",ret);
builder.setPositiveButton("确定" , null );
builder.show();
}else{
AlertDialog.Builder builder = new AlertDialog.Builder(MeetingInfoDetailActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("出现异常,请重试!") ;
String ret = ""+Longitude + Latitude;
Log.d("ret = ",ret);
builder.setPositiveButton("确定" , null );
builder.show();
}
} catch (JSONException e) {
e.printStackTrace();
//statuTV.setText("签到异常");
}
}
});
}
});
}
private void scanCodeSign(String meetingId){
RequestBody requestBodyPost = new FormBody.Builder()
.add("meetingId",meetingId)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.DeleteMeeting)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
JSONObject jsObj = new JSONObject(retStr);
if (jsObj.getString("melodyClass").equals("yes")){
AlertDialog.Builder builder = new AlertDialog.Builder(MeetingInfoDetailActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("会议删除成功!" ) ;
builder.setPositiveButton("确定" , new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which)
{
// 设置TextView文本
//点击是的时候去进行提交
Intent intent = new Intent(MeetingInfoDetailActivity.this,AdministrationActivity.class);
intent.putExtra("teacherId",teacherId);
startActivity(intent);
}
} );
builder.show();
}else {
AlertDialog.Builder builder = new AlertDialog.Builder(MeetingInfoDetailActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("会议删除失败!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}
} catch (JSONException e) {
e.printStackTrace();
//statuTV.setText("签到异常");
}
}
});
}
});
}
}
<file_sep>package com.xinnuo.apple.nongda.studentActivity.OnlineTest;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.LinearLayout;
import android.widget.TextView;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class ApplyForExemptionActivity extends AppCompatActivity {
private OkHttpClient client;
private LinearLayout Layout_application; //添加申请
private LinearLayout apply_layout; //申请列表
private TextView stuName; //学生姓名
private TextView stuNo; //学生编号
private TextView stuTime; //申请时间
private TextView stuState; //状态(1、成功 2、否 3、申请中 4、拒绝)
private Button apply_withdraw; //取消申请
private String id;
private String name;
private String studentNo;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_apply_for_exemption);
initOkHttp();
binding();
final Intent intent = getIntent();
id = intent.getStringExtra("id");
name = intent.getStringExtra("name");
studentNo = intent.getStringExtra("stuNo");
apply_withdraw.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
final AlertDialog.Builder builder = new AlertDialog.Builder(ApplyForExemptionActivity.this);
builder.setMessage("是否确认取消申请!").
// 设置确定按钮
setPositiveButton("确定",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which)
{
// 设置TextView文本
//点击是的时候去进行提交
scanCodeSign1("2");
}
}).
// 设置取消按钮
setNegativeButton("取消",
new DialogInterface.OnClickListener()
{
public void onClick(DialogInterface dialog,
int which)
{
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
}
});
Layout_application.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent1 = new Intent(ApplyForExemptionActivity.this,AddApplicationActivity.class);
intent1.putExtra("name",name);
intent1.putExtra("studentNo",studentNo);
intent1.putExtra("id",id);
startActivity(intent1);
}
});
}
private void binding()
{
Layout_application = (LinearLayout) findViewById(R.id.Layout_application);
apply_layout = (LinearLayout) findViewById(R.id.apply_layout);
stuName = (TextView) findViewById(R.id.apply_stuName);
stuNo = (TextView) findViewById(R.id.apply_stuNo);
stuTime = (TextView) findViewById(R.id.apply_time);
stuState = (TextView) findViewById(R.id.apply_state);
apply_withdraw = (Button) findViewById(R.id.apply_withdraw);
}
public void onStart(){
super.onStart();
scanCodeSign();
}
//添加或取消申请免试
private void scanCodeSign1(String states){
RequestBody requestBodyPost = new FormBody.Builder()
.add("studentNo",studentNo)
.add("exemprtionStatus",states)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.AddApplication)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
JSONObject jsObj = new JSONObject(retStr);
String retStr = jsObj.getString("melodyClass");
if (retStr.equals("yes")){
scanCodeSign();
Layout_application.setVisibility(View.VISIBLE);
apply_layout.setVisibility(View.VISIBLE);
apply_withdraw.setVisibility(View.INVISIBLE);
}else{
}
Layout_application.setVisibility(View.VISIBLE);
apply_layout.setVisibility(View.GONE);
} catch (JSONException e) {
e.printStackTrace();
//statuTV.setText("签到异常");
}
}
});
}
});
}
private void scanCodeSign(){
RequestBody requestBodyPost = new FormBody.Builder()
.add("id",id)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.ApplyForExemptionQueery)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
apply_layout.setVisibility(View.VISIBLE);
Layout_application.setVisibility(View.GONE);
JSONArray jsArray = new JSONArray(retStr);
for (int i = 0 ; i < jsArray.length() ; i++){
JSONObject jsObj1 = jsArray.getJSONObject(i);
stuName.setText(jsObj1.getString("name"));
stuNo.setText(studentNo);
stuTime.setText(jsObj1.getString("time"));
String states = null;
if (jsObj1.getString("state").equals("1")){
states = "成功";
}else if(jsObj1.getString("state").equals("2")){
states = "否";
Layout_application.setVisibility(View.VISIBLE);
}else if (jsObj1.getString("state").equals("3")){
states = "申请中";
apply_withdraw.setVisibility(View.VISIBLE);
}else if (jsObj1.getString("state").equals("4")){
states = "拒绝";
}
stuState.setText(states);
}
} catch (JSONException e) {
e.printStackTrace();
JSONObject jsObj = null;
try {
jsObj = new JSONObject(retStr);
String retStr = jsObj.getString("melodyClass");
Layout_application.setVisibility(View.VISIBLE);
apply_layout.setVisibility(View.GONE);
} catch (JSONException e1) {
e1.printStackTrace();
}
//statuTV.setText("签到异常");
}
}
});
}
});
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda;
import android.app.Activity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.RadioGroup;
import android.widget.TextView;
import com.amap.api.location.AMapLocation;
import com.amap.api.location.AMapLocationClient;
import com.amap.api.location.AMapLocationClientOption;
import com.amap.api.location.AMapLocationClientOption.AMapLocationMode;
import com.amap.api.location.AMapLocationClientOption.AMapLocationProtocol;
import com.amap.api.location.AMapLocationListener;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
/**
* 高精度定位模式功能演示
*
* @创建时间: 2015年11月24日 下午5:22:42
* @项目名称: AMapLocationDemo2.x
* @author hongming.wang
* @文件名称: Hight_Accuracy_Activity.java
* @类型名称: Hight_Accuracy_Activity
*/
public class Location_Activity extends Activity implements View.OnClickListener {
private RadioGroup rgLocationMode;
private EditText etInterval;
private EditText etHttpTimeout;
private CheckBox cbOnceLocation;
private CheckBox cbAddress;
private CheckBox cbGpsFirst;
private CheckBox cbCacheAble;
private CheckBox cbOnceLastest;
private CheckBox cbSensorAble;
private TextView statuTV;
private Button btLocation;
private static double signLongitude;
private static double signLatitude;
private AMapLocationClient locationClient = null;
private AMapLocationClientOption locationOption = new AMapLocationClientOption();
private static final double ALERT_DISTANCE = 50;
private OkHttpClient client;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_location);
btLocation = (Button) findViewById(R.id.bt_location);
statuTV = (TextView) findViewById(R.id.textView);
btLocation.setOnClickListener(this);
//初始化定位
initLocation();
}
@Override
protected void onDestroy() {
super.onDestroy();
destroyLocation();
}
/**
* 设置控件的可用状态
*/
private void setViewEnable(boolean isEnable) {
for(int i=0; i<rgLocationMode.getChildCount(); i++){
rgLocationMode.getChildAt(i).setEnabled(isEnable);
}
etInterval.setEnabled(isEnable);
etHttpTimeout.setEnabled(isEnable);
cbOnceLocation.setEnabled(isEnable);
cbGpsFirst.setEnabled(isEnable);
cbAddress.setEnabled(isEnable);
cbCacheAble.setEnabled(isEnable);
cbOnceLastest.setEnabled(isEnable);
cbSensorAble.setEnabled(isEnable);
}
/**
* 初始化定位
*
* @since 2.8.0
* @author hongming.wang
*
*/
private void initLocation(){
//初始化client
locationClient = new AMapLocationClient(this.getApplicationContext());
//设置定位参数
locationClient.setLocationOption(getDefaultOption());
// 设置定位监听
locationClient.setLocationListener(locationListener);
}
/**
* 默认的定位参数
* @since 2.8.0
* @author hongming.wang
*
*/
private AMapLocationClientOption getDefaultOption(){
AMapLocationClientOption mOption = new AMapLocationClientOption();
mOption.setLocationMode(AMapLocationMode.Hight_Accuracy);//可选,设置定位模式,可选的模式有高精度、仅设备、仅网络。默认为高精度模式
mOption.setGpsFirst(false);//可选,设置是否gps优先,只在高精度模式下有效。默认关闭
mOption.setHttpTimeOut(30000);//可选,设置网络请求超时时间。默认为30秒。在仅设备模式下无效
mOption.setInterval(2000);//可选,设置定位间隔。默认为2秒
mOption.setNeedAddress(true);//可选,设置是否返回逆地理地址信息。默认是true
mOption.setOnceLocation(false);//可选,设置是否单次定位。默认是false
mOption.setOnceLocationLatest(false);//可选,设置是否等待wifi刷新,默认为false.如果设置为true,会自动变为单次定位,持续定位时不要使用
AMapLocationClientOption.setLocationProtocol(AMapLocationProtocol.HTTP);//可选, 设置网络请求的协议。可选HTTP或者HTTPS。默认为HTTP
//mOption.setSensorEnable(false);//可选,设置是否使用传感器。默认是false
return mOption;
}
/**
* 定位监听
*/
AMapLocationListener locationListener = new AMapLocationListener() {
@Override
public void onLocationChanged(AMapLocation loc) {
if (null != loc) {
//解析定位结果
String result = Utils.getLocationStr(loc);
/**
* sb.append("经 度 : " + location.getLongitude() + "\n");
sb.append("纬 度 : " + location.getLatitude() + "\n");
*/
double Longitude = loc.getLongitude();
double Latitude = loc.getLatitude();
String ret = ""+Longitude + Latitude;
String jStr = Longitude+",";
String wStr = Latitude+"";
statuTV.setText("定位结果:long = "+Longitude+"lat = "+Latitude);
// test3TV.setText("定位结果:long = "+Longitude+"lat = "+Latitude);
double distance = DistanceOfTwoPoints(Latitude,Longitude,signLatitude,signLongitude);
// test4TV.setText("计算结果"+distance);
// if (distance > ALERT_DISTANCE)
// {
// statuTV.setText("签到失败。距离定位点"+distance+"米");
//// test5TV.setText("超出距离");
//
// }
// else
// {
// //立即签到
// signRequest();
//// test5TV.setText("在距离内");
//
// }
//
} else {
statuTV.setText("定位失败,loc is null");
}
}
};
/**
* 开始定位
*
* @since 2.8.0
* @author hongming.wang
*
*/
private void startLocation(){
// 设置定位参数
locationClient.setLocationOption(locationOption);
// 启动定位
locationClient.startLocation();
}
/**
* 停止定位
*
* @since 2.8.0
* @author hongming.wang
*
*/
private void stopLocation(){
// 停止定位
locationClient.stopLocation();
}
/**
* 销毁定位
*
* @since 2.8.0
* @author hongming.wang
*
*/
private void destroyLocation(){
if (null != locationClient) {
/**
* 如果AMapLocationClient是在当前Activity实例化的,
* 在Activity的onDestroy中一定要执行AMapLocationClient的onDestroy
*/
locationClient.onDestroy();
locationClient = null;
locationOption = null;
}
}
@Override
public void onClick(View v) {
statuTV.setText("正在定位...");
startLocation();
}
/**
* 根据两点间经纬度坐标(double值),计算两点间距离,
*
* @param lat1
* @param lng1
* @param lat2
* @param lng2
* @return 距离:单位为米
*/
public static double DistanceOfTwoPoints(double lat1,double lng1,
double lat2,double lng2) {
double radLat1 = rad(lat1);
double radLat2 = rad(lat2);
double a = radLat1 - radLat2;
double b = rad(lng1) - rad(lng2);
double s = 2 * Math.asin(Math.sqrt(Math.pow(Math.sin(a / 2), 2)
+ Math.cos(radLat1) * Math.cos(radLat2)
* Math.pow(Math.sin(b / 2), 2)));
s = s * 6378137.0;
s = Math.round(s * 10000) / 10000;
return s;
}
private void signRequest(){
RequestBody requestBodyPost = new FormBody.Builder()
//.add("id", userId)
.build();
Request requestPost = new Request.Builder()
//.url(makeSignSleep)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
JSONObject jsObj = new JSONObject(retStr);
String retStr = jsObj.getString("melodyClass");
if (retStr.equals("yes")) {
statuTV.setText("签到成功");
}
else if (retStr.equals("no"))
{
statuTV.setText("不是签到时间");
}
else if (retStr.equals("guiqin"))
{
statuTV.setText("已经签到过了");
}else{
statuTV.setText("服务器异常");
}
stopLocation();
} catch (JSONException e) {
e.printStackTrace();
statuTV.setText("签到异常");
stopLocation();
}
}
});
}
});
}
private static double rad(double d) {
return d * Math.PI / 180.0;
}
}
<file_sep>package com.xinnuo.apple.nongda.studentActivity.TeacherEvaluation;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class TeacherScoreActivity extends BaseActivity {
private TextView core1;
private TextView core2;
private TextView core3;
private TextView core4;
private TextView core5;
private TextView core6,core7;
private Button coreButton;
private String core;
private String teacherId;
private String id;
private OkHttpClient client;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_teacher_score);
initOkHttp();
Intent intent = getIntent();
teacherId = intent.getStringExtra("teacherId");
id = intent.getStringExtra("id");
core1 = (TextView) findViewById(R.id.core1);
core2 = (TextView) findViewById(R.id.core2);
core3 = (TextView) findViewById(R.id.core3);
core4 = (TextView) findViewById(R.id.core4);
core5 = (TextView) findViewById(R.id.core5);
core6 = (TextView) findViewById(R.id.core6);
core7 = (TextView) findViewById(R.id.core7);
coreButton = (Button) findViewById(R.id.core_button);
core2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
core = "0";
core1.setText(core);
}
});
core3.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
core = "2";
core1.setText(core);
}
});
core4.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
core = "2";
core1.setText(core);
}
});
core5.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
core = "3";
core1.setText(core);
}
});
core6.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
core = "4";
core1.setText(core);
}
});
core7.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
core = "5";
core1.setText(core);
}
});
coreButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 设置显示信息
final AlertDialog.Builder builder = new AlertDialog.Builder(TeacherScoreActivity.this);
builder.setMessage("确定要提交?").
// 设置确定按钮
setPositiveButton("是",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which)
{
// 设置TextView文本
//点击是的时候去进行提交
requestWithUserId();
}
}).
// 设置取消按钮
setNegativeButton("否",
new DialogInterface.OnClickListener()
{
public void onClick(DialogInterface dialog,
int which)
{
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
}
});
}
/**
* 请求方法
* */
private void requestWithUserId(){
//菊花的开始方法
mLoading.show();
RequestBody requestBodyPost = new FormBody.Builder()
.add("teacherId",teacherId)
.add("score",core)
.add("id",id)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.AddTeacherEvaluation)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
mLoading.dismiss();
e.printStackTrace();
}
}
});
}
});
}
/**
* 解析数据方法
* */
private void jsonParseWithJsonStr (String jsonStr) throws JSONException {
JSONArray jsArr = new JSONArray(jsonStr);
List<String> data = new ArrayList<String>();
ArrayList<HashMap<String, Object>> listItem = new ArrayList<HashMap<String, Object>>();/*在数组中存放数据*/
for (int i = 0; i < jsArr.length(); i++)
{
JSONObject js = jsArr.getJSONObject(i);
String relTime = js.optString("relTime");
String meetType;
if (js.getString("melodyClass").equals("成功"))
{
AlertDialog.Builder builder = new AlertDialog.Builder(TeacherScoreActivity.this);
builder.setTitle("提示");
builder.setMessage("评价上传成功!");
builder.setPositiveButton("确定",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which)
{
// 设置TextView文本
//点击是的时候去进行提交
if (core.length() == 0){
AlertDialog.Builder builder = new AlertDialog.Builder(TeacherScoreActivity.this);
builder.setTitle("提示");
builder.setMessage("请选择分数!");
builder.setPositiveButton("确定", null);
builder.show();
}else {
Intent intent = new Intent(TeacherScoreActivity.this,TeacherEvaluationActivity.class);
intent.putExtra("id",id);
startActivity(intent);
}
}
});
builder.show();
}else
{
AlertDialog.Builder builder = new AlertDialog.Builder(TeacherScoreActivity.this);
builder.setTitle("提示");
builder.setMessage("评价上传失败!");
builder.setPositiveButton("确定", null);
builder.show();
}
teacherId = js.getString("id");
}
if (jsArr.length() == 0)
{
midToast("无数据",3);
}
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda;
import android.app.AlertDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.telephony.TelephonyManager;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import com.xinnuo.apple.nongda.Admin.AdminActivity;
import com.xinnuo.apple.nongda.Binding.StudentBindingActivity;
import com.xinnuo.apple.nongda.Binding.TeacherBindingActivity;
import com.xinnuo.apple.nongda.entity.AdminModel;
import com.xinnuo.apple.nongda.entity.StudentModel;
import com.xinnuo.apple.nongda.entity.TeacherModel;
import com.xinnuo.apple.nongda.singin.Student_Singin;
import com.xinnuo.apple.nongda.singin.Teacher_Singin;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import cn.jpush.android.api.JPushInterface;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class MainActivity extends BaseActivity {
private EditText phone_editText;
private EditText pswd_editText;
private CheckBox pswd_checkBox;
private Button login_button;
private Button Retrieve_button;
private OkHttpClient client;
SharedPreferences sp = null;
private static final int REQUEST_CODE_SCAN = 0x0000;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
sp = getSharedPreferences("userinfo",Context.MODE_PRIVATE);
initView();
initOkHttp();
//自动登录 若选中为true 自动登录 不勾选为fouse
if (sp.getBoolean("pswd_checkBox", true)) {
phone_editText.setText(sp.getString("uname", null));
pswd_editText.setText(sp.getString("upswd", null));
//设置为勾选
pswd_checkBox.setChecked(true);
//取出记住的账号和密码
String name = phone_editText.getText().toString().trim();
String pswd = pswd_editText.getText().toString().trim();
//若不为空自动登录
if (name.length() > 0 && pswd.length() > 0) {
//调用请求方法进行请求
onClieck(name, pswd);
}
} else {
//若为空 将checkboxButton设置为false
pswd_checkBox.setChecked(false);
}
}
//绑定控件
private void initView() {
phone_editText = (EditText) findViewById(R.id.phone_editext);
pswd_editText = (EditText) findViewById(R.id.pswd_editText);
pswd_checkBox = (CheckBox) findViewById(R.id.pswd_checkBox);
login_button = (Button) findViewById(R.id.login_button);
Retrieve_button = (Button) findViewById(R.id.Retrieve_button);
LimitsEditEnter(phone_editText);
login_button.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View v) {
if (v == login_button) {
mLoading.show();
//取出用户输入的账号和密码
final String name = phone_editText.getText().toString().trim();
String pswd = pswd_editText.getText().toString().trim();
//判断进行相应的弹窗提示
if (name.equals("")) {
midToast("用户名空", 1);
mLoading.dismiss();
return;
}
if (pswd.equals("")) {
midToast("密码空", 1);
mLoading.dismiss();
return;
}
//查看CheckBoxLogin是否勾选上
boolean CheckBoxLogin = pswd_checkBox.isChecked();
if (CheckBoxLogin) {
SharedPreferences.Editor editor = sp.edit();
editor.putString("uname", name);
editor.putString("upswd", pswd);
editor.putBoolean("checkboxBoolean", true);
editor.commit();
} else {
SharedPreferences.Editor editor = sp.edit();
editor.putString("uname", null);
editor.putString("upswd", null);
editor.putBoolean("checkboxBoolean", false);
editor.commit();
}
// //判断输入的账号格式是否正确 正确进行跳转 不正确给出提示信息 并跳出此方法
// if (IsPhoneNumUtil.isMobileNumber(name))
// {
// Log.d("phone","phonenumber格式正确");
// }else
// { //菊花的结束方法
// mLoading.dismiss();
// midToast("不是电信号段", 1);
// return;
// }
//请求的登录方法
onClieck(name, pswd);
}
}
});
}
//请求方法 参数手机号 密码
private void onClieck(String userId, String password) {
TelephonyManager TelephonyMgr = (TelephonyManager) getSystemService(TELEPHONY_SERVICE);
String szImei = "";//TelephonyMgr.getDeviceId();
String rid = JPushInterface.getRegistrationID(getApplicationContext());
String phoneType = android.os.Build.MODEL;
RequestBody requestBodyPost = new FormBody.Builder()
.add("name", userId)
.add("password", <PASSWORD>)
.add("registrationID", rid)//极光推送的id 手机类型
.add("deviceNumber", szImei)//绑定的手机序列号
.add("phoneType",phoneType)//手机的产品名字
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.loginUrl)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
Log.d("TAG", "网络请求 = onFailure: "+e);
// midToast("登录异常:error = 3", 1);
mLoading.dismiss();
}
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值", retStr);
//菊花结束方法
mLoading.dismiss();
try {
//调用解析方法解析数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
e.printStackTrace();
mLoading.dismiss();
//提示信息
midToast("登录异常:error = 1", 1);
}
}
});
}
});
}
//解析数据对象
private void jsonParseWithJsonStr(String jsonStr) throws JSONException {
Student_Singin student_singin = new Student_Singin();
Teacher_Singin teacher_singin = new Teacher_Singin();
StudentModel studentModel = new StudentModel();
TeacherModel teacherModel = new TeacherModel();
JSONArray js = new JSONArray(jsonStr);
for (int i = 0; i < js.length(); i++)
{
JSONObject jsObj = js.getJSONObject(i);
Log.d("******","********");
String loginSta = jsObj.getString("loginSta");
Log.d("******",loginSta);
/**
* loginSta:
* fail 失败
* success 教师登录成功
* successToStu 学生登录成功
* formation 立即验证学生
* teacherFormation 立即验证教师
* deviceNumber 不是绑定设备
*
*/
//fail 失败
if (loginSta.equals("fail"))
{
midToast("用户名或密码错误", 1);
}
//success 学生登录成功
else if (loginSta.equals("successToStu"))
{
/**
* status:
* status=1 学生
* status=2 管理员
* status=3 教师
* */
int status = jsObj.getInt("status");
Log.d("******",status+"");
//学生登录
if (status == 1)
{
//为学生对象赋值
studentModel = student_singin.setSutdentInfoWith(jsObj);
//调用跳转方法返回intent
Intent intent = student_singin.stu_Login(MainActivity.this,jsObj,studentModel);
//启动跳转
startActivity(intent);
finish();
}
}else if (loginSta.equals("success"))
{
int status = jsObj.getInt("status");
Log.d("******",status+"");
if (status == 3)
{
midToast("登陆成功",3);
teacherModel = teacher_singin.setTeacherInfo(jsObj);
Intent intent = teacher_singin.teacher_Login(MainActivity.this,jsObj,teacherModel);
intent.putExtra("cardNo",teacherModel.getCardNo());
intent.putExtra("pswd",teacherModel.getPassword());
intent.putExtra("phone",teacherModel.getPhone());
startActivity(intent);
finish();
}else if (status == 2)
{
/**
*
* "birthday": "null",
"phone": "123456",
"sex": 1,
"status": 2,
"jurisdiction": "1",
"collegeName": "null",
"password": "<PASSWORD>",
"id": 67,
"cardNo": "null",
"picture": "03.jpg",
"name": "admin",
"itro": "null",
"loginSta": "success"*/
AdminModel adminModel = new AdminModel();
adminModel.setBirthday(jsObj.optString("birthday"));
adminModel.setPhone(jsObj.optString("phone"));
adminModel.setSex(jsObj.getInt("sex"));
adminModel.setStatus(jsObj.getInt("status"));
adminModel.setJurisdiction(jsObj.optString("jurisdiction"));
adminModel.setCollegeName(jsObj.optString("collegeName"));
adminModel.setPassword(jsObj.getString("password"));
adminModel.setId(jsObj.getString("id"));
adminModel.setCardNo(jsObj.getString("cardNo"));
adminModel.setPicture(jsObj.getString("picture"));
adminModel.setItro(jsObj.getString("itro"));
adminModel.setLoginSta(jsObj.getString("loginSta"));
adminModel.setName(jsObj.getString("name"));
Intent intent = new Intent(MainActivity.this,AdminActivity.class);
intent.putExtra("adminName",jsObj.getString("name"));
intent.putExtra("adminId",jsObj.getString("id"));
startActivity(intent);
finish();
}
}else if (loginSta.equals("formation"))
{
final AlertDialog.Builder builder = new AlertDialog.Builder(this);
final String stuNo = phone_editText.getText().toString().trim();
Log.d("*****",stuNo);
// 设置显示信息
builder.setMessage("身份未绑定,是否绑定?").
// 设置确定按钮
setPositiveButton("是",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which) {
//进行页面跳转 跳到绑定界面需要输入身份证号和手机号进行绑定
Intent intent = new Intent(MainActivity.this, StudentBindingActivity.class);
//传值学生id
intent.putExtra("studentNo", stuNo);
String state = "1";
intent.putExtra("state",state);
startActivity(intent);
finish();
}
}).
// 设置取消按钮
setNegativeButton("否",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
}else if (loginSta.equals("teacherFormation"))
{
final AlertDialog.Builder builder = new AlertDialog.Builder(this);
final String userNo = phone_editText.getText().toString().trim();
//取出教师id
final String teacherBindId = jsObj.getString("id");
// 设置显示信息
builder.setMessage("身份未绑定,是否绑定?").
// 设置确定按钮
setPositiveButton("是",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which) {
//点击是进行页面跳转,输入教师工号和手机号进行绑定
Intent intent = new Intent(MainActivity.this, TeacherBindingActivity.class);
//传值 教师id
intent.putExtra("teacherInfoId", teacherBindId);
String state = "1";
intent.putExtra("state",state);
startActivity(intent);
finish();
}
}).
// 设置取消按钮
setNegativeButton("否",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
}
}
}
//初始化请求
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda.studentActivity.StudentResultQuery;
/**
* 学生成绩查询
* */
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import android.widget.TextView;
import com.xinnuo.apple.nongda.R;
public class StudentResultQueryActivity extends AppCompatActivity {
private TextView student_query1;
private TextView student_query2;
private TextView student_query3;
private TextView student_query4;
private String id; //学生Id
private String studentNo; //学号
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_student_result_query);
binding();
clickJump();
Intent intent = getIntent();
id = intent.getStringExtra("id");
studentNo = intent.getStringExtra("studentNo");
Log.d("=============",studentNo);
}
/**
* 绑定控件
* */
protected void binding()
{
student_query1 = (TextView) findViewById(R.id.student_query1);
student_query2 = (TextView) findViewById(R.id.student_query2);
student_query3 = (TextView) findViewById(R.id.student_query3);
student_query4 = (TextView) findViewById(R.id.student_query4);
}
/**
* 点击进行相应的跳转
* */
protected void clickJump()
{
//签到记录查询
student_query1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(StudentResultQueryActivity.this,StuAttendanceRecordActivity.class);
intent.putExtra("id",id);
intent.putExtra("studentNo",studentNo);
startActivity(intent);
}
});
//体质成绩查询
student_query2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(StudentResultQueryActivity.this,PhysicalPerformanceActivity.class);
intent.putExtra("id",id);
startActivity(intent);
}
});
//在线考试成绩查询
student_query3.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(StudentResultQueryActivity.this,OnlineAnswerQueryActivity.class);
intent.putExtra("id",id);
intent.putExtra("studentNo",studentNo);
startActivity(intent);
}
});
//体育成绩查询
student_query4.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(StudentResultQueryActivity.this,SportsPerformanceQueryActivity.class);
intent.putExtra("studentNo",studentNo);
startActivity(intent);
}
});
}
}
<file_sep>package com.xinnuo.apple.nongda.Admin;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import android.widget.TextView;
import com.xinnuo.apple.nongda.Admin.Club.AdminClub;
import com.xinnuo.apple.nongda.Admin.Meeting.AdminMeetingInfoActivity;
import com.xinnuo.apple.nongda.Admin.News.AdminNewsActivity;
import com.xinnuo.apple.nongda.Admin.Substitute.AdminSubstituteActivity;
import com.xinnuo.apple.nongda.MainActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.PrintStream;
import java.util.Scanner;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class AdminActivity extends AppCompatActivity {
//定义控件
private TextView admin_meeting;
private TextView admin_spase;
private TextView admin_spase1;
private TextView admin_achievement;
private TextView admin_setup;
private TextView admin_Replace;
private TextView admin_check;
private TextView admin_club;
private TextView admin_name;
private OkHttpClient client;
private String adminId;
private final static String FILE_NAME = "xth.txt"; // 设置文件的名称
private String spDate;
private String adminName;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_admin);
boundControl();
Intent intent = getIntent();
adminName = intent.getStringExtra("adminName");
adminId = intent.getStringExtra("adminId");
admin_name.setText(adminName);
assignBtnAction();
}
/**
* 绑定控件
* */
private void boundControl()
{
admin_name = (TextView) findViewById(R.id.admin_name);
admin_club = (TextView) findViewById(R.id.admin_club);
admin_check = (TextView) findViewById(R.id.admin_check);
admin_achievement = (TextView) findViewById(R.id.admin_achievement);
admin_Replace = (TextView) findViewById(R.id.admin_Replace);
admin_setup = (TextView) findViewById(R.id.admin_setup);
admin_meeting = (TextView) findViewById(R.id.admin_meeting);
admin_spase = (TextView) findViewById(R.id.admin_spase);
admin_spase1 = (TextView) findViewById(R.id.admin_spase1s);
}
/**
* 点击事件方法 点击相应的功能进行相应的跳转并进行传值
* */
private void assignBtnAction()
{
//俱乐部
admin_club.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view)
{
Intent intent = new Intent(AdminActivity.this, AdminClub.class);
String statu = "0";
intent.putExtra("statu",statu);
startActivity(intent);
}
});
//考勤数据统计
admin_check.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view)
{
Intent intent = new Intent(AdminActivity.this, AdminClub.class);
String statu = "1";
intent.putExtra("statu",statu);
startActivity(intent);
}
});
//成绩查询
admin_achievement.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view)
{
Intent intent = new Intent(AdminActivity.this, AdminClub.class);
String statu = "2";
intent.putExtra("statu",statu);
startActivity(intent);
}
});
//会议及集体活动
admin_meeting.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(AdminActivity.this, AdminMeetingInfoActivity.class);
intent.putExtra("teacherId",adminId);
startActivity(intent);
}
});
//账号设置
admin_setup.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
loginOut();
}
});
//代课
admin_Replace.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(AdminActivity.this, AdminSubstituteActivity.class);
intent.putExtra("teacherId",adminId);
startActivity(intent);
}
});
//消息
admin_spase.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
SharedPreferences preferences=getSharedPreferences("userinfo",Context.MODE_PRIVATE);
SharedPreferences.Editor editor=preferences.edit();
editor.putString("msgDate", spDate);
editor.commit();
Intent intent = new Intent(AdminActivity.this,AdminNewsActivity.class);
startActivity(intent);
}
});
}
@Override
protected void onResume() {
super.onResume();
findNewMsg();
}
//查询是否是最新消息
private void findNewMsg(){
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
RequestBody requestBodyPost = new FormBody.Builder()
.add("status",1+"")
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.StuNews)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
public static final String TAG = "admin";
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
//{"msgDate":"2017-01-18 15:27:40","msgContent":"张海里荣升一只鸡\n"}
JSONObject jsObj = new JSONObject(retStr);
String msgDate = jsObj.getString("msgDate");
spDate = msgDate;
SharedPreferences preferences=getSharedPreferences("userinfo", Context.MODE_PRIVATE);
String saveDate=preferences.getString("msgDate", null);
Log.d(TAG, "run: msgDate"+msgDate);
Log.d(TAG, "run: savaDate"+saveDate);
if (msgDate.equals(saveDate))
{
Log.d(TAG, "run: 相同");
admin_spase1.setVisibility(View.INVISIBLE);
}else
{
Log.d(TAG, "run: 不相同");
admin_spase1.setVisibility(View.VISIBLE);
}
} catch (JSONException e) {
e.printStackTrace();
//statuTV.setText("签到异常");
}
}
});
}
});
}
private StringBuffer read() {
FileInputStream in = null;
Scanner s = null;
StringBuffer sb = new StringBuffer();
try {
in = super.openFileInput(FILE_NAME);
s = new Scanner(in);
while (s.hasNext()) {
sb.append(s.next());
}
} catch (Exception e) {
e.printStackTrace();
}
return sb;
}
private void save(String data) {
FileOutputStream out = null;
PrintStream ps = null;
try {
out = super.openFileOutput(FILE_NAME, Activity.MODE_APPEND);
ps = new PrintStream(out);
ps.println(data);
} catch (Exception e) {
e.printStackTrace();
} finally {
if (out != null) {
try {
out.close();
ps.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
//退出方法 点击注销时给出提示信息
private void loginOut(){
// 设置显示信息
final AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage("确定要退出?").
// 设置确定按钮
setPositiveButton("是",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which) {
// 设置TextView文本
//点击是的时候去进行提交
SharedPreferences sp = getSharedPreferences("userinfo",
Context.MODE_PRIVATE);
SharedPreferences.Editor editor = sp.edit();
editor.putBoolean("pswd_checkBox", false);
editor.commit();
//进行页面跳转,跳回登录界面
Intent intent = new Intent(AdminActivity.this, MainActivity.class);
startActivity(intent);
finish();
}
}).
// 取消跳转
setNegativeButton("否",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
}
}
<file_sep>package com.xinnuo.apple.nongda.Admin.ResultQuery;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.TextView;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.TeacherActivity.Resultquery.TeacherClassQueryActivity;
public class AdminResultQueryActivity extends AppCompatActivity {
private TextView attendanceRecord;
private TextView constitutionQuery;
private TextView onlineAnswer;
private TextView sportsPerformance;
private String teacherId;
private String itemId;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_admin_result_query);
Intent intent = getIntent();
teacherId = intent.getStringExtra("id");
itemId = intent.getStringExtra("itemId");
BoundControl();
PageJump();
}
/**
*绑定控件方法
* */
private void BoundControl()
{
attendanceRecord = (TextView) findViewById(R.id.admin_query1);
constitutionQuery = (TextView) findViewById(R.id.admin_query2);
onlineAnswer = (TextView) findViewById(R.id.admin_query3);
sportsPerformance = (TextView) findViewById(R.id.admin_query4);
}
/**
* 点击事件
* 点击相应的TextView进行页面跳转
* */
private void PageJump()
{ //签到记录查询
attendanceRecord.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view)
{
String state = "1";
Intent intent = new Intent(AdminResultQueryActivity.this,TeacherClassQueryActivity.class);
intent.putExtra("teacherId",teacherId);
intent.putExtra("itemId",itemId);
intent.putExtra("state",state);
startActivity(intent);
}
});
//体质测试查询
constitutionQuery.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view)
{
String state = "2";
Intent intent = new Intent(AdminResultQueryActivity.this,TeacherClassQueryActivity.class);
intent.putExtra("teacherId",teacherId);
intent.putExtra("itemId",itemId);
intent.putExtra("state",state);
startActivity(intent);
}
});
//在线答题成绩查询
onlineAnswer.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view)
{
String state = "3";
Intent intent = new Intent(AdminResultQueryActivity.this,TeacherClassQueryActivity.class);
intent.putExtra("teacherId",teacherId);
intent.putExtra("itemId",itemId);
intent.putExtra("state",state);
startActivity(intent);
}
});
//体育成绩查询
sportsPerformance.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view)
{
String state = "4";
Intent intent = new Intent(AdminResultQueryActivity.this,TeacherClassQueryActivity.class);
intent.putExtra("teacherId",teacherId);
intent.putExtra("itemId",itemId);
intent.putExtra("state",state);
startActivity(intent);
}
});
}
}
<file_sep>package com.xinnuo.apple.nongda.TeacherActivity.AttendanceDataStatistics;
/**
* 教师考勤记录 0离职 1未离职
* */
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.util.Log;
import android.view.KeyEvent;
import android.view.View;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.RelativeLayout;
import android.widget.TextView;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import com.xinnuo.apple.nongda.view.XListView;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class AttendanceDataStatisticsActivity extends BaseActivity implements XListView.IXListViewListener {
private OkHttpClient client;
private String teacherId;
private int page;
private ArrayList<HashMap<String, Object>> dlist;
private boolean flag = false;
private ArrayAdapter<String> mAdapter;
private int number = 0;
private Handler mHandler;
private ListView listView;
private boolean isFirstIn = true;
private RelativeLayout mHeaderViewContent;
private TextView tea_attendance_record; //考勤情况
private TextView tea_turnover_record; //离职情况
private TextView tea_tendance_times; //考勤次数及离职次数
private XListView mListView;
private ArrayList<String> items;
private ArrayList<String> items1;
private String state;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_attendance_data_statistics);
Intent intent = getIntent();
teacherId = intent.getStringExtra("teacherId");
tea_attendance_record = (TextView) findViewById(R.id.tea_attendance_record);
tea_turnover_record = (TextView) findViewById(R.id.tea_turnover_record);
tea_tendance_times = (TextView) findViewById(R.id.tea_number);
initOkHttp();
tea_attendance_record.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
items.clear();
state = "1";
number = 0;
requestWithDataAdd(0,state);
}
});
tea_turnover_record.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
items.clear();
state = "0";
number = 0;
requestWithDataAdd(0,state);
}
});
}
protected void onStart()
{
super.onStart();
state = "1";
requestWithDataAdd(0,state);
mListView = (XListView) findViewById(R.id.xListView);
mListView.setPullLoadEnable(true);
mAdapter = new ArrayAdapter<String>(AttendanceDataStatisticsActivity.this, R.layout.item_attendance_data_sta, items);
mListView.setAdapter(mAdapter);
mListView.setXListViewListener(AttendanceDataStatisticsActivity.this);
mHandler = new Handler();
}
/**
* 网络请求
* @param add add = 0 刷新 add = 1 加载更多
*/
private void requestWithDataAdd(int add,String state){
if (add == 0)
{
page = 1;
items = new ArrayList<String>();
}
else
{
page ++;
}
//菊花的开始方法
mLoading.show();
RequestBody requestBodyPost = new FormBody.Builder()
.add("teacherid",teacherId)
.add("pageNumber",page+"")
.add("state",state)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.AttendanceDataStatistics)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
mLoading.dismiss();
e.printStackTrace();
}
}
});
}
});
}
/**
* 解析数据方法
* 0 离职 1未离职
* */
//json解析方法
private void jsonParseWithJsonStr (String jsonStr) throws JSONException {
try{
JSONArray jsArr = null;
String state = null;
if ((!jsonStr.equals("[]")) || (!jsonStr.equals("{\"nul\":\"\"}")))
{
jsArr = new JSONArray(jsonStr);
String currName = null;
String attendEndDate = null;
for (int i = 0; i < jsArr.length(); i++)
{
JSONObject js = jsArr.getJSONObject(i);
state = js.getString("state");
if (state.equals("0"))
{
JSONArray jss = js.getJSONArray("date");
number ++;
for (int j = 0 ; j < jss.length(); j++)
{
JSONObject jsonObject = jss.getJSONObject(j);
currName = "离职时间:" + jsonObject.getString("DepartureTime");
tea_tendance_times.setText("考勤:"+number+"次");
items.add(currName);
}
}else
{
JSONArray jss = js.getJSONArray("date");
number ++;
for (int j = 0 ; j < jss.length(); j++)
{
JSONObject jsonObject = jss.getJSONObject(j);
currName = "上课时间:" + jsonObject.getString("attendDate");
attendEndDate = "下课时间:"+ jsonObject.getString("attendEndDate");
String s = currName + "\n" +attendEndDate;
tea_tendance_times.setText("考勤:"+number+"次");
items.add(s);
}
}
}
}else if (jsonStr.equals("{\"nul\":\"\"}")){
midToast("无数据",3);
}else {
midToast("无数据",3);
}
mAdapter = new ArrayAdapter<String>(AttendanceDataStatisticsActivity.this, R.layout.item_attendance_data_sta, items);
mListView.setAdapter(mAdapter);
mAdapter.notifyDataSetChanged();
onLoad();
}catch (Exception e)
{
midToast("无数据",3);
return;
}
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
@Override
public boolean onKeyDown(int keyCode, KeyEvent event) {
if (keyCode == KeyEvent.KEYCODE_BACK && event.getRepeatCount() == 0) {
this.finish();
}
return false;
}
@Override
public void onRefresh() {
mHandler.postDelayed(new Runnable() {
@Override
public void run() {
if (state.equals("0"))
{
requestWithDataAdd(1,state);
onLoad();
}else
{
requestWithDataAdd(1,state);
onLoad();
}
}
}, 2000);
}
private void onLoad() {
mListView.stopRefresh();
mListView.stopLoadMore();
mListView.setRefreshTime("刚刚");
}
@Override
public void onLoadMore() {
mHandler.postDelayed(new Runnable() {
@Override
public void run() {
if (state.equals("0"))
{
requestWithDataAdd(page,state);
onLoad();
}else
{
requestWithDataAdd(page,state);
onLoad();
}
}
}, 2000);
}
}
<file_sep>package com.xinnuo.apple.nongda.TeacherActivity.Resultquery;
/**
* 班级展示
* */
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class StudentListQueryActivity extends BaseActivity {
private OkHttpClient client;
private String classId;
private String itemId;
private ListView listView;
private String state;
private JSONArray dataArr;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_student_list_query);
initOkHttp();
listView = (ListView) findViewById(R.id.list_stuquerys);
Intent intent = getIntent();
classId = intent.getStringExtra("id");
itemId = intent.getStringExtra("itemId");
state = intent.getStringExtra("state");
if (state .equals("1"))
{
requestWithUserId(itemId,classId, httpUrl.studentListUrl);
}else if(state.equals("2"))
{
requestWithUserId(itemId,classId, httpUrl.StudentPhysicalFitnessScore);
}else if (state.equals("3"))
{
requestWithUserId(itemId,classId, httpUrl.SportsClassIdQueryStudentsOnline);
}else
{
requestWithUserId(itemId,classId, httpUrl.TeacherSportsPerformanceQuery);
}
}
/**
* 请求方法
* */
private void requestWithUserId(String itemId,String id,String url){
//菊花的开始方法
mLoading.show();
RequestBody requestBodyPost = new FormBody.Builder()
.add("classId",id)
.add("sportclassId",id)
.add("itemId",itemId)
.build();
Request requestPost = new Request.Builder()
.url(url)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr,state);
} catch (JSONException e) {
mLoading.dismiss();
e.printStackTrace();
}
}
});
}
});
}
/**
* 解析数据方法
* */
//json解析方法
private void jsonParseWithJsonStr (String jsonStr , String state) throws JSONException {
JSONArray jsArr = new JSONArray(jsonStr);
dataArr = jsArr;
List<String> data = new ArrayList<String>();
ArrayList<HashMap<String, Object>> listItem = new ArrayList<HashMap<String, Object>>();/*在数组中存放数据*/
if (state .equals("1"))
{
HashMap<String, Object> map2 = new HashMap<String, Object>();
map2.put("count","序 号");
map2.put("studentNo"," 学 号");
map2.put("stuname","姓 名");
map2.put("number","次 数");
map2.put("state",state);
listItem.add(map2);
for (int i = 0; i < jsArr.length(); i++) {
JSONObject js = jsArr.getJSONObject(i);
/*
* "studentNo": "11042227 ",
* "name": "张烁",
* "number": 0
*/
String count = i+1+"";
String studentNo = js.optString("studentNo");
String stuname = js.optString("name");
String number = js.optString("number");
Log.d("******",studentNo);
HashMap<String, Object> map = new HashMap<String, Object>();
// map.put("id",id);
map.put("count",count);
map.put("studentNo",studentNo);
map.put("stuname",stuname);
map.put("number",number);
listItem.add(map);
}
}else if (state.equals("2")) {
HashMap<String, Object> map2 = new HashMap<String, Object>();
map2.put("count", "序 号");
map2.put("studentNo", " 学 号");
map2.put("stuname", "姓 名");
map2.put("number", "总 分");
listItem.add(map2);
for (int i = 0; i < jsArr.length(); i++) {
JSONObject js = jsArr.getJSONObject(i);
/*
* "studentNo": "11042227 ",
* "name": "张烁",
* "number": 0
*/
String count = i + 1 + "";
String studentNo = js.optString("studentId");
String stuname = js.optString("name");
String number = js.optString("result");
Log.d("******", studentNo);
HashMap<String, Object> map = new HashMap<String, Object>();
// map.put("id",id);
map.put("count", count);
map.put("studentNo", studentNo);
map.put("stuname", stuname);
map.put("number", number);
listItem.add(map);
}
}else if (state.equals("4")){
HashMap<String, Object> map2 = new HashMap<String, Object>();
map2.put("count", "序 号");
map2.put("studentNo", " 学 号");
map2.put("stuname", "姓 名");
map2.put("number", "体育成绩");
listItem.add(map2);
for (int i = 0; i < jsArr.length(); i++) {
JSONObject js = jsArr.getJSONObject(i);
/*
* "studentNo": "11042227 ",
* "name": "张烁",
* "number": 0
*/
String count = i + 1 + "";
String studentNo = js.optString("studentNo");
String stuname = js.optString("name");
String number = js.optString("zongFen");
Log.d("******", studentNo);
HashMap<String, Object> map = new HashMap<String, Object>();
// map.put("id",id);
map.put("count", count);
map.put("studentNo", studentNo);
map.put("stuname", stuname);
map.put("number", number);
listItem.add(map);
}
}else if (state.equals("3")){
HashMap<String, Object> map2 = new HashMap<String, Object>();
map2.put("count", "序 号");
map2.put("studentNo", " 学 号");
map2.put("stuname", "姓 名");
map2.put("number", "分 数");
listItem.add(map2);
for (int i = 0; i < jsArr.length(); i++) {
JSONObject js = jsArr.getJSONObject(i);
/*
* "studentNo": "11042227 ",
* "name": "张烁",
* "number": 0
*/
String count = i + 1 + "";
String studentNo = js.optString("studentNo");
String stuname = js.optString("name");
String number = js.optString("stuScore");
Log.d("******", studentNo);
HashMap<String, Object> map = new HashMap<String, Object>();
// map.put("id",id);
map.put("count", count);
map.put("studentNo", studentNo);
map.put("stuname", stuname);
map.put("number", number);
listItem.add(map);
}
}
if (jsArr.length() == 0)
{
midToast("无数据",3);
}
SimpleAdapter qrAdapter = new SimpleAdapter(this,listItem, R.layout.item_student_list_query,
new String[]{"count","studentNo","stuname","number"},
new int[]{R.id.studentlist_query1, R.id.studentlist_query2, R.id.studentlist_query3, R.id.studentlist_query4});
listView.setAdapter(qrAdapter);
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda.studentActivity.AccountSettings;
/**
* 修改密码
* */
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class StuModifyPasswordActivity extends BaseActivity implements View.OnClickListener{
private OkHttpClient client;
private EditText stu_old_pswd;
private EditText stu_new_pswd;
private EditText stu_confirm_pswd;
private Button stu_button_pswd;
private String id;
private String studentNo;
private String password;
private String new_paswd;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_stu_modify_password);
Intent intent = getIntent();
id = intent.getStringExtra("id");
studentNo = intent.getStringExtra("studentNo");
Log.d("stuNo = ",studentNo);
password = intent.getStringExtra("password");
initOkHttp();
binding();
}
//绑定控件
private void binding()
{
stu_old_pswd = (EditText) findViewById(R.id.stu_old_pswd);
stu_new_pswd = (EditText) findViewById(R.id.stu_new_pswd);
stu_confirm_pswd = (EditText) findViewById(R.id.stu_confirm_pswd);
stu_button_pswd = (Button) findViewById(R.id.stu_button_pswd);
stu_button_pswd.setOnClickListener(this);
}
/**
* 请求方法
* */
private void requestWithUserId(String id, String studentNos ,String oldpswd,String newPswds){
//菊花的开始方法
mLoading.show();
RequestBody requestBodyPost = new FormBody.Builder()
.add("id",id)
.add("studentNo",studentNo)
.add("topas",oldpswd)
.add("pwdByStu",newPswds)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.StudentModifyPassword)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
mLoading.dismiss();
e.printStackTrace();
}
}
});
}
});
}
/**
* 解析数据方法
* */
//json解析方法
private void jsonParseWithJsonStr (String jsonStr) throws JSONException {
JSONObject js = new JSONObject(jsonStr);
if (js.getString("melodyClass").equals("yes"))
{
AlertDialog.Builder builder = new AlertDialog.Builder(StuModifyPasswordActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("修改成功!" ) ;
builder.setPositiveButton("确定" , new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which)
{
// 设置TextView文本
//进行跳转
Intent intent = new Intent(StuModifyPasswordActivity.this,StuAccountSettingsActivity.class);
intent.putExtra("id",id);
intent.putExtra("studentNo",studentNo);
intent.putExtra("password",<PASSWORD>);
startActivity(intent);
}
}) ;
builder.show();
}else
{
AlertDialog.Builder builder = new AlertDialog.Builder(StuModifyPasswordActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("修改失败,请重新提交!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
@Override
public void onClick(View view) {
new_paswd = stu_new_pswd.getText().toString();
if (new_paswd.length() >= 6) {
String confirmPswd = stu_confirm_pswd.getText().toString();
if (new_paswd.equals(confirmPswd)) {
// 设置显示信息
final AlertDialog.Builder builder = new AlertDialog.Builder(StuModifyPasswordActivity.this);
builder.setMessage("确定要提交?").
// 设置确定按钮
setPositiveButton("是",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which) {
// 设置TextView文本
//点击是的时候去进行提交
requestWithUserId(id, studentNo, password,new_paswd);
}
}).
// 设置取消按钮
setNegativeButton("否",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
} else {
AlertDialog.Builder builder = new AlertDialog.Builder(StuModifyPasswordActivity.this);
builder.setTitle("提示");
builder.setMessage("两次输入的密码不一致,请重新输入!");
builder.setPositiveButton("确定", null);
builder.show();
}
}else {
AlertDialog.Builder builder = new AlertDialog.Builder(StuModifyPasswordActivity.this);
builder.setTitle("提示");
builder.setMessage("密码长度必须大于6位,请重新输入!");
builder.setPositiveButton("确定", null);
builder.show();
}
}
}
<file_sep>package com.xinnuo.apple.nongda.TeacherActivity.SportsStandards;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class Sport_Class extends BaseActivity {
private OkHttpClient client;
private String id;
private String sportkindSex;
private String sportkindName;
private String status;
private ListView listView;
private JSONArray dataArr;
private String state;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_sport__class);
initOkHttp();
listView = (ListView) findViewById(R.id.list_stu_sport_class);
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long is) {
try {
if (state.equals("2")){
//点击相应的班级进行跳转
Intent intent=new Intent(Sport_Class.this,SportsScore.class);
JSONObject js = dataArr.getJSONObject(position);
//传值 班级id 所教班级id
intent.putExtra("id",id);
intent.putExtra("sex",js.getString("sex"));
intent.putExtra("studentId",js.getString("id"));
intent.putExtra("studentNo",js.getString("studentNo"));
intent.putExtra("name",js.getString("name"));
intent.putExtra("sportkindSex",sportkindSex);
intent.putExtra("sportkindName",sportkindName);
intent.putExtra("status",status);
startActivity(intent);
finish();
}else{
Intent intent=new Intent(Sport_Class.this,SportSecondActivity.class);
intent.putExtra("status",status);
startActivity(intent);
finish();
}
} catch (JSONException e) {
e.printStackTrace();
}
}
});
}
protected void onStart()
{
super.onStart();
//取出上个界面传过来的id
Intent intent = getIntent();
id = intent.getStringExtra("id");
sportkindSex = intent.getStringExtra("sportkindSex");
sportkindName = intent.getStringExtra("sportkindName");
status = intent.getStringExtra("status");
state = intent.getStringExtra("state");
Log.d("*****",status+"*****************************");
requestWithUserId(id);
}
//请求方法
private void requestWithUserId(String id){
mLoading.show();
Log.d("StudentList ==== ", id);
RequestBody requestBodyPost = new FormBody.Builder()
.add("id",id) //id
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.TeacherClubStudentNumberUrl) //请求地址
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收后台返回值方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
e.printStackTrace();
}
}
});
}
});
}
//json解析
private void jsonParseWithJsonStr (String jsonStr) throws JSONException {
JSONArray jsArr = new JSONArray(jsonStr);
dataArr = jsArr;
ArrayList<HashMap<String, Object>> listItem = new ArrayList<HashMap<String, Object>>();/*在数组中存放数据*/
HashMap<String, Object> map2 = new HashMap<String, Object>();
map2.put("Item1","序 号");
map2.put("Item2"," 学 号");
map2.put("Item3","姓 名");
map2.put("Item4","班 级");
map2.put("Item5","性 别");
listItem.add(map2);
/*
* "id": 42960,
"sex": "1",(1 = 男 、2 = 女)
"studentNo": "14011411",
"gradeId": 2,
"name": "姜博",
"className": "汉语14-4",
"collegeName": "人文学院"*/
for (int i = 0; i < jsArr.length(); i++) {
String jsStr = jsArr.getString(i);
JSONObject js = jsArr.getJSONObject(i);
HashMap<String, Object> map = new HashMap<String, Object>();
map.put("Item1",i+1);
map.put("Item2", js.getString("studentNo"));
map.put("Item3", js.getString("name"));
map.put("Item4", js.getString("collegeName")+"\n"+js.getString("className"));
String sex = null;
if (js.getString("sex").equals("1")){
sex = "男";
map.put("Item5", sex);
} else{
sex = "女";
map.put("Item5", sex);
}
if (sportkindSex.equals(sex)){
listItem.add(map);
}
}
if (jsArr.length() == 0)
{
midToast("无数据",3);
}
SimpleAdapter qrAdapter = new SimpleAdapter(this,listItem, R.layout.item_teacher_classsize,
new String[]{"Item1","Item2","Item3","Item4","Item5"},
new int[]{R.id.teacher_stunumber1, R.id.teacher_stunumber2, R.id.teacher_stunumber3, R.id.teacher_stunumber4, R.id.teacher_stunumber5});
listView.setAdapter(qrAdapter);
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda.TeacherActivity.SportsStandards;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class SportsDetails extends BaseActivity {
private String id;
private OkHttpClient client;
private ListView listView;
private JSONArray dataArr;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_sports_details);
initOkHttp();
Intent intent = getIntent();
id = intent.getStringExtra("id");
listView= (ListView)findViewById(R.id.list_sport_details);
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long is) {
try {
//点击相应的班级进行跳转
JSONObject js = dataArr.getJSONObject(position);
if (js.getString("sportkindName").equals("50米跑(秒)") || js.getString("sportkindName").equals("1000米跑") || js.getString("sportkindName").equals("800米跑") )
{
//传值 班级id 所教班级id
Intent intent=new Intent(SportsDetails.this,RunActivity.class);
intent.putExtra("id",id);
intent.putExtra("sportkindSex",js.getString("sportkindSex"));
intent.putExtra("sportkindName",js.getString("sportkindName"));
intent.putExtra("state","1");
String status = position+1+"";
intent.putExtra("status",status);
startActivity(intent);
}else{
//传值 班级id 所教班级id
Intent intent=new Intent(SportsDetails.this,Sport_Class.class);
intent.putExtra("id",id);
intent.putExtra("sportkindSex",js.getString("sportkindSex"));
intent.putExtra("sportkindName",js.getString("sportkindName"));
intent.putExtra("state","2");
String status = position+1+"";
intent.putExtra("status",status);
startActivity(intent);
}
} catch (JSONException e) {
e.printStackTrace();
}
}
});
}
public void onStart(){
super.onStart();
requestWithUserId();
}
/**
* 请求方法
* */
private void requestWithUserId(){
//菊花的开始方法
mLoading.show();
RequestBody requestBodyPost = new FormBody.Builder()
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.SportsDetails)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
mLoading.dismiss();
e.printStackTrace();
}
}
});
}
});
}
/**
* 解析数据方法
* */
//json解析方法
private void jsonParseWithJsonStr (String jsonStr) throws JSONException {
JSONArray jsArr = new JSONArray(jsonStr);
dataArr = jsArr;
List<String> data = new ArrayList<String>();
ArrayList<HashMap<String, Object>> listItem = new ArrayList<HashMap<String, Object>>();/*在数组中存放数据*/
for (int i = 0; i < jsArr.length(); i++) {
JSONObject jss = jsArr.getJSONObject(i);
String sportkindName = jss.getString("sportkindName");
String sportkindSex = jss.optString("sportkindSex");
HashMap<String, Object> map = new HashMap<String, Object>();
// ap.put("id",id);
map.put("sportkindName",sportkindName);
map.put("sportkindSex",sportkindSex);
listItem.add(map);
}
if (jsArr.length() == 0)
{
midToast("无数据",3);
}
SimpleAdapter qrAdapter = new SimpleAdapter(this,listItem, R.layout.item_sports_details,
new String[]{"sportkindName","sportkindSex"},
new int[]{R.id.sport_detail1, R.id.sport_detail2});
listView.setAdapter(qrAdapter);
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda.Admin.Meeting;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.EditText;
import android.widget.LinearLayout;
import android.widget.TextView;
import android.widget.Toast;
import com.xinnuo.apple.nongda.Admin.widget.PickTimeView;
import com.xinnuo.apple.nongda.Admin.widget.PickValueView;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class NewReleaseActivity extends BaseActivity implements View.OnClickListener, PickTimeView.onSelectedChangeListener, PickValueView.onSelectedChangeListener {
private EditText title;
private TextView oject;
private TextView times;
private EditText place;
private EditText introduction;
private TextView immediate_release;
private String[] strings;
private int count = 0;
private int state = 0;
private OkHttpClient client;
private String titles;
private String places;
private String introductions;
private String invitationState;
private String invitationTeachers;
private String pushState;
LinearLayout pvLayout;
PickTimeView pickTime;
PickTimeView pickDate;
PickValueView pickValue;
PickValueView pickValues;
PickValueView pickString;
SimpleDateFormat sdfTime;
SimpleDateFormat sdfDate;
private String meetingTime;
private String teacherId;
private String titles1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_new_release);
binding();
initOkHttp();
Intent intent = getIntent();
if (intent.getStringArrayExtra("string") != null){
strings = intent.getStringArrayExtra("string");
invitationState = "2";
for (int i = 0; i < strings.length; i++){
if(i == 0) invitationTeachers = strings[i];
else invitationTeachers += "," + strings[i];
Log.d("==============",invitationTeachers+strings[i]+"****************");
}
Log.d("==============",invitationTeachers+"****************");
oject.setText("从名单选择");
}else{
invitationState = "1";
invitationTeachers = "0";
oject.setText("学校全体教师");
}
if (intent.getStringExtra("teacherId") != null){
teacherId = intent.getStringExtra("teacherId");
}
if (intent.getStringExtra("titles1") != null){
title.setText(intent.getStringExtra("titles1"));
}
}
private void binding(){
title = (EditText) findViewById(R.id.admin_title);
oject = (TextView) findViewById(R.id.admin_object);
times = (TextView) findViewById(R.id.admin_meeting_time);
place = (EditText) findViewById(R.id.admin_meeting_place);
introduction = (EditText) findViewById(R.id.admin_meeting_introduction);
immediate_release = (TextView) findViewById(R.id.immediate_release);
pvLayout = (LinearLayout) findViewById(R.id.Main_pvLayout);
pickTime = (PickTimeView) findViewById(R.id.pickTime);
pickDate = (PickTimeView) findViewById(R.id.pickDate);
pickValue = (PickValueView) findViewById(R.id.pickValue);
pickValues = (PickValueView) findViewById(R.id.pickValues);
pickString = (PickValueView) findViewById(R.id.pickString);
sdfTime = new SimpleDateFormat("MM-dd EEE HH:mm");
sdfDate = new SimpleDateFormat("yyyy-MM-dd");
pickTime.setOnSelectedChangeListener(this);
pickDate.setOnSelectedChangeListener(this);
pickValue.setOnSelectedChangeListener(this);
pickValues.setOnSelectedChangeListener(this);
pickString.setOnSelectedChangeListener(this);
title.setOnClickListener(this);
oject.setOnClickListener(this);
times.setOnClickListener(this);
place.setOnClickListener(this);
introduction.setOnClickListener(this);
immediate_release.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()){
case R.id.admin_object:
final String[] items = new String[]{"学校全体教师","从名单选择","取消"};
final AlertDialog.Builder builder = new AlertDialog.Builder(NewReleaseActivity.this);
builder.setItems(items, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
switch (which) {
case 0:
Toast.makeText(NewReleaseActivity.this, "您选中了:"+items[0], Toast.LENGTH_SHORT).show();
oject.setText(items[0]);
state = 1;
invitationState = "1";
invitationTeachers = "0";
break;
case 1:
Toast.makeText(NewReleaseActivity.this, "您选中了:"+items[1], Toast.LENGTH_SHORT).show();
Intent intent2 = new Intent(NewReleaseActivity.this, AdminQueryAllteacherActivity.class);
intent2.putExtra("teacherId",teacherId);
intent2.putExtra("titles",title.getText().toString());
startActivity(intent2);
oject.setText(items[1]);
state = 1;
break;
case 2:
Toast.makeText(NewReleaseActivity.this, "您选中了:"+items[2], Toast.LENGTH_SHORT).show();
break;
}
}
}).show();
break;
case R.id.admin_meeting_time:
if (count == 0){
pvLayout.setVisibility(View.VISIBLE);
count++;
}else {
pvLayout.setVisibility(View.GONE);
count = 0;
}
break;
case R.id.immediate_release:
titles = title.getText().toString();
places = place.getText().toString();
introductions = introduction.getText().toString();
if (titles.length() == 0 ){
AlertDialog.Builder builders = new AlertDialog.Builder(NewReleaseActivity.this);
builders.setTitle("提示" ) ;
builders.setMessage("请补全信息!" ) ;
builders.setPositiveButton("确定" , null );
builders.show();
}else if (places.length() == 0){
AlertDialog.Builder builders = new AlertDialog.Builder(NewReleaseActivity.this);
builders.setTitle("提示" ) ;
builders.setMessage("请补全信息!" ) ;
builders.setPositiveButton("确定" , null );
builders.show();
}else if (introductions.length() == 0){
AlertDialog.Builder builders = new AlertDialog.Builder(NewReleaseActivity.this);
builders.setTitle("提示" ) ;
builders.setMessage("请补全信息!" ) ;
builders.setPositiveButton("确定" , null );
builders.show();
}else{
final String[] itemss = new String[]{"发布,并通知相关人员","发布但不通知","取消"};
final AlertDialog.Builder builder1 = new AlertDialog.Builder(NewReleaseActivity.this);
builder1.setItems(itemss, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
switch (which) {
case 0:
pushState = "0";
scanCodeSign(pushState);
break;
case 1:
pushState = "1";
scanCodeSign(pushState);
break;
case 2:
Toast.makeText(NewReleaseActivity.this, "您选中了:"+itemss[2], Toast.LENGTH_SHORT).show();
break;
}
}
}).show();
}
break;
}
}
@Override
public void onSelected(PickTimeView view, long timeMillis) {
if (view == pickTime) {
String str = sdfTime.format(timeMillis);
times.setText(str);
meetingTime = str;
} else if (view == pickDate) {
String str = sdfDate.format(timeMillis);
times.setText(str);
meetingTime = str;
}
}
@Override
public void onSelected(PickValueView view, Object leftValue, Object middleValue, Object rightValue) {
if (view == pickValue) {
int left = (int) leftValue;
times.setText("selected:" + left);
} else if (view == pickValues) {
int left = (int) leftValue;
int middle = (int) middleValue;
int right = (int) rightValue;
times.setText("selected: left:" + left + " middle:" + middle + " right:" + right);
} else {
String selectedStr = (String) leftValue;
times.setText(selectedStr);
}
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
private void scanCodeSign(String pushState){
RequestBody requestBodyPost = new FormBody.Builder()
.add("meetingTime",meetingTime)
.add("id",teacherId)
.add("place",places)
.add("introduction",introductions)
.add("theme",titles)
.add("invitationState",invitationState)
.add("invitationTeachers",invitationTeachers)
.add("pushState",pushState)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.AddMeeting)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
JSONObject jsObj = new JSONObject(retStr);
if (jsObj.getString("melodyClass").equals("yes")){
AlertDialog.Builder builder = new AlertDialog.Builder(NewReleaseActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("会议发布成功!" ) ;
builder.setPositiveButton("确定" , new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which)
{
// 设置TextView文本
//点击是的时候去进行提交
Intent intent = new Intent(NewReleaseActivity.this,AdministrationActivity.class);
intent.putExtra("teacherId",teacherId);
startActivity(intent);
finish();
}
} );
builder.show();
}else {
AlertDialog.Builder builder = new AlertDialog.Builder(NewReleaseActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("会议发布失败!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}
} catch (JSONException e) {
e.printStackTrace();
//statuTV.setText("签到异常");
}
}
});
}
});
}
}
<file_sep>package com.xinnuo.apple.nongda.TeacherActivity.AccountSettings;
/**
* 修改密码
* */
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class ModifyPasswordActivity extends BaseActivity implements View.OnClickListener{
private OkHttpClient client;
private EditText old_pswd;
private EditText new_pswd;
private EditText confirm_pswd;
private Button button_pswd;
private String cardNo;
private String phone;
private String teacherId;
private String newPswd;
private String oldPswd;
private String pswd;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_modify_password);
setTitle("农大 => 修改密码");
binding();
initOkHttp();
Intent intent = getIntent();
cardNo = intent.getStringExtra("cardNo");
Log.d("cardNo = ", cardNo);
phone = intent.getStringExtra("phone");
teacherId = intent.getStringExtra("teacherId");
pswd = intent.getStringExtra("pswd");
}
//绑定控件
private void binding()
{
old_pswd = (EditText) findViewById(R.id.old_pswd);
new_pswd = (EditText) findViewById(R.id.new_pswd);
confirm_pswd = (EditText) findViewById(R.id.confirm_pswd);
button_pswd = (Button) findViewById(R.id.button_pswd);
button_pswd.setOnClickListener(this);
}
/**
* 请求方法
* */
private void requestWithUserId(String cardNos, String phones ,String newPswds){
//菊花的开始方法
mLoading.show();
RequestBody requestBodyPost = new FormBody.Builder()
.add("cardNo",cardNo)
.add("phone",phone)
.add("password",<PASSWORD>)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.TeacherModifyPassword)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
mLoading.dismiss();
e.printStackTrace();
}
}
});
}
});
}
/**
* 解析数据方法
* */
//json解析方法
private void jsonParseWithJsonStr (String jsonStr) throws JSONException {
JSONObject js = new JSONObject(jsonStr);
if (js.getString("melodyClass").equals("操作成功"))
{
AlertDialog.Builder builder = new AlertDialog.Builder(ModifyPasswordActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("修改成功!" ) ;
builder.setPositiveButton("确定" , new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which)
{
// 设置TextView文本
//进行跳转
Intent intent = new Intent(ModifyPasswordActivity.this,AccountSettingsActivity.class);
intent.putExtra("cardNo",cardNo);
intent.putExtra("phone",phone);
intent.putExtra("teacherId",teacherId);
startActivity(intent);
finish();
}
}) ;
builder.show();
}else
{
AlertDialog.Builder builder = new AlertDialog.Builder(ModifyPasswordActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("修改失败,请重新提交!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
@Override
public void onClick(View view) {
oldPswd = old_pswd.getText().toString();
newPswd = new_pswd.getText().toString();
if (oldPswd.equals(pswd)){
if (newPswd.length() > 6) {
String confirmPswd = confirm_pswd.getText().toString();
if (newPswd.equals(confirmPswd)) {
// 设置显示信息
final AlertDialog.Builder builder = new AlertDialog.Builder(ModifyPasswordActivity.this);
builder.setMessage("确定要提交?").
// 设置确定按钮
setPositiveButton("是",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which) {
// 设置TextView文本
//点击是的时候去进行提交
requestWithUserId(cardNo, phone, newPswd);
}
}).
// 设置取消按钮
setNegativeButton("否",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
} else {
AlertDialog.Builder builder = new AlertDialog.Builder(ModifyPasswordActivity.this);
builder.setTitle("提示");
builder.setMessage("两次输入的密码不一致,请重新输入!");
builder.setPositiveButton("确定", null);
builder.show();
}
}else {
AlertDialog.Builder builder = new AlertDialog.Builder(ModifyPasswordActivity.this);
builder.setTitle("提示");
builder.setMessage("密码长度必须大于6位,请重新输入!");
builder.setPositiveButton("确定", null);
builder.show();
}
}else {
AlertDialog.Builder builder = new AlertDialog.Builder(ModifyPasswordActivity.this);
builder.setTitle("提示");
builder.setMessage("旧密码输入不正确,请重新输入!");
builder.setPositiveButton("确定", null);
builder.show();
}
}
}
<file_sep>package com.xinnuo.apple.nongda.Admin.News;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import android.widget.TextView;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import com.xinnuo.apple.nongda.studentActivity.News.MessageDetails;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class AdminNewsActivity extends BaseActivity {
private OkHttpClient client;
private ListView listView;
private JSONArray jsonArray;
private TextView adminNews;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_admin_news);
initOkHttp();
listView = (ListView) findViewById(R.id.list_admin_news);
adminNews = (TextView) findViewById(R.id.admin_news);
scanCodeSign();
listView.setOnItemClickListener(new AdapterView.OnItemClickListener(){
public void onItemClick(AdapterView<?> parent, View view, int position, long id)
{
try {
JSONObject jsonObject = jsonArray.getJSONObject(position);
Intent intent = new Intent(AdminNewsActivity.this,MessageDetails.class);
intent.putExtra("time",jsonObject.getString("msgDate"));
intent.putExtra("content",jsonObject.getString("msgContent"));
startActivity(intent);
} catch (JSONException e) {
e.printStackTrace();
}
}
});
adminNews.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(AdminNewsActivity.this,ReleaseMessageActivity.class);
startActivity(intent);
}
});
}
private void scanCodeSign(){
RequestBody requestBodyPost = new FormBody.Builder()
.add("status",2+"")
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.StuNews)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
JSONArray jsa = new JSONArray(retStr);
jsonArray = jsa;
ArrayList<HashMap<String, Object>> listItem = new ArrayList<HashMap<String, Object>>();/*在数组中存放数据*/
for (int i = 0 ; i < jsa.length() ; i++)
{
JSONObject jsonObject = jsa.getJSONObject(i);
String masgDate = jsonObject.getString("msgDate");
String msgContent = jsonObject.getString("msgContent");
HashMap<String, Object> map = new HashMap<String, Object>();
map.put("masgDate",masgDate);
map.put("msgContent",msgContent);
listItem.add(map);
}
if (jsa.length() == 0)
{
midToast("无数据",3);
}
SimpleAdapter qrAdapter = new SimpleAdapter(AdminNewsActivity.this,listItem, R.layout.item_news,
new String[]{"masgDate","msgContent"},
new int[]{R.id.stu_news_time, R.id.stu_news_content});
listView.setAdapter(qrAdapter);
} catch (JSONException e) {
e.printStackTrace();
//statuTV.setText("签到异常");
}
}
});
}
});
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda.TeacherActivity.CourseAttendance;
/**
* e二维码签到
* */
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.Color;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.telephony.TelephonyManager;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.WriterException;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import java.io.IOException;
import java.util.Random;
import java.util.concurrent.TimeUnit;
import cn.jpush.android.api.JPushInterface;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class TeacherMakeQRActivity extends AppCompatActivity {
private OkHttpClient client;
private String sportClassId;
private String itemId;
private String teacherId;
private Button refreshBtn;
private ImageView imageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_teacher_make_qr);
initOkHttp();
//绑定 控件
refreshBtn = (Button)findViewById(R.id.refreshBtn);
imageView = (ImageView) findViewById(R.id.code_image);
//取出教师id 所教课程id
Intent intent = getIntent();
teacherId = intent.getStringExtra("teacherId");
itemId = intent.getStringExtra("itemId");
sportClassId = intent.getStringExtra("sportClassId");
makeQRImg();
refreshBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
makeQRImg();
}
});
}
private void makeQRImg()
{
String QRNumber = getRandomString(10);
Bitmap qrcode = generateQRCode(teacherId+itemId+sportClassId+","+QRNumber);
upLoadQR(QRNumber,"1");
imageView.setImageBitmap(qrcode);
}
private void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
private Bitmap bitMatrix2Bitmap(BitMatrix matrix) {
int w = matrix.getWidth();
int h = matrix.getHeight();
int[] rawData = new int[w * h];
for (int i = 0; i < w; i++) {
for (int j = 0; j < h; j++) {
int color = Color.WHITE;
if (matrix.get(i, j)) {
color = Color.BLACK;
}
rawData[i + (j * w)] = color;
}
}
Bitmap bitmap = Bitmap.createBitmap(w, h, Bitmap.Config.RGB_565);
bitmap.setPixels(rawData, 0, w, 0, 0, w, h);
return bitmap;
}
//生成二维码 参数教师id 教师所教课程id
private Bitmap generateQRCode(String content) {
try {
QRCodeWriter writer = new QRCodeWriter();
// MultiFormatWriter writer = new MultiFormatWriter();
BitMatrix matrix = writer.encode(content, BarcodeFormat.QR_CODE, 700, 700);
return bitMatrix2Bitmap(matrix);
} catch (WriterException e) {
e.printStackTrace();
}
return null;
}
public static String getRandomString(int length) { //length表示生成字符串的长度
String base = "abcdefghijklmnopqrstuvwxyz0123456789";
Random random = new Random();
StringBuffer sb = new StringBuffer();
for (int i = 0; i < length; i++) {
int number = random.nextInt(base.length());
sb.append(base.charAt(number));
}
return sb.toString();
}
private void upLoadQR(String theKey, String status) {
Log.d("000", "onDestroy:++++++++++++++++++++++++++ ");
TelephonyManager TelephonyMgr = (TelephonyManager) getSystemService(TELEPHONY_SERVICE);
String szImei = TelephonyMgr.getDeviceId();
String rid = JPushInterface.getRegistrationID(getApplicationContext());
String phoneType = android.os.Build.MODEL;
RequestBody requestBodyPost = new FormBody.Builder()
.add("teacherid", teacherId)
.add("status",status)
.add("theKey", theKey)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.TeacherUpdateTheQrCodeKey)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
Log.d("000", "onFailure:++++++++++++++++++++++++++ ");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值", retStr);
}
});
}
});
}
}
<file_sep>package com.xinnuo.apple.nongda.TeacherActivity.CourseAttendance;
/**
* 签到主界面 二维码 定位 上课 下课 合课
* */
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.LinearLayout;
import android.widget.TextView;
import com.amap.api.location.AMapLocation;
import com.amap.api.location.AMapLocationClient;
import com.amap.api.location.AMapLocationClientOption;
import com.amap.api.location.AMapLocationListener;
import com.xinnuo.apple.nongda.Anticlockwise;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.Utils;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class ClassListDetailsActivity extends BaseActivity {
private TextView scan_code_sign; //扫码签到
private TextView opening_positioning; //开启定位
private TextView close_location; //关闭定位
private TextView Attendance_list; //考勤列表
private TextView combined_lesson; //合课
private TextView course_start; //课程开始
private TextView end_of_course; //课程结束
private TextView refresh_position; //刷新位置
private OkHttpClient client;
private String sportclassId;
private static double Longitude;
private static double Latitude;
private String teacherId;
private Anticlockwise mTimer;
private LinearLayout layouts;
private String classState;
private JSONObject datrr;
private String itemId;
private static double distance;
private String coordinateId; //坐标id
private LinearLayout layout;
private boolean flag;
private AMapLocationClient locationClient = null;
private AMapLocationClientOption locationOption = new AMapLocationClientOption();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_class_list_details);
binding();
initOkHttp();
Intent intent = getIntent();
sportclassId = intent.getStringExtra("sportclassId");
Log.d("sportclassId = ",sportclassId);
teacherId = intent.getStringExtra("teacherId");
itemId = intent.getStringExtra("itemId");
//初始化时间
clickJump();
}
protected void onStart()
{
super.onStart();
}
//绑定控件
private void binding()
{
scan_code_sign = (TextView) findViewById(R.id.scan_code_sign);
opening_positioning = (TextView) findViewById(R.id.opening_positioning);
close_location = (TextView) findViewById(R.id.close_location);
Attendance_list = (TextView) findViewById(R.id.Attendance_list);
combined_lesson = (TextView) findViewById(R.id.combined_lesson);
course_start = (TextView) findViewById(R.id.course_start);
end_of_course = (TextView) findViewById(R.id.end_of_course);
refresh_position = (TextView) findViewById(R.id.refresh_position);
layout = (LinearLayout) findViewById(R.id.layout_effect);
mTimer = (Anticlockwise) findViewById(R.id.id_timer);
}
//点击事件
private void clickJump()
{
//扫码签到
scan_code_sign.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view) {
Intent intent = new Intent(ClassListDetailsActivity.this,TeacherMakeQRActivity.class);
intent.putExtra("teacherId",teacherId);
intent.putExtra("sportClassId",sportclassId);
intent.putExtra("itemId",itemId);
startActivity(intent);
}
});
//开启定位
opening_positioning.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view) {
String open = "1";
startAndClose(open);
Log.d("********","走的这里"+Latitude+"Latitude"+Latitude);
}
});
//关闭定位
close_location.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view) {
if (Longitude == 0)
{
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("定位失败请重新定位,然后再关闭定位!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}else {
String close = "2";
startAndClose(close);
}
}
});
//考勤列表
Attendance_list.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view) {
Intent intent = new Intent(ClassListDetailsActivity.this,TeacherAttendanceListActivity.class);
intent.putExtra("sportclassId",sportclassId);
startActivity(intent);
}
});
//合课
combined_lesson.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view) {
if (Latitude == 0 && Latitude == 0)
{
//statuTV.setText("不是签到时间");
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("请先开启定位!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}else
{
Intent intent = new Intent(ClassListDetailsActivity.this,CombinedLessonActivity.class);
intent.putExtra("coordinateId",coordinateId);
intent.putExtra("sportclassId1",sportclassId);
intent.putExtra("teacherId",teacherId);
startActivity(intent);
}
}
});
//课程开始
course_start.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view) {
if (distance > 50){
mTimer.reStart();
}
signRequest();
}
});
//课程结束
end_of_course.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view) {
endOfCourse();
}
});
}
private void initLocation(){
//初始化client
locationClient = new AMapLocationClient(this.getApplicationContext());
//设置定位参数
locationClient.setLocationOption(getDefaultOption());
// 设置定位监听
locationClient.setLocationListener(locationListener);
}
/**
* 开始定位
* @since 2.8.0
* @author hongming.wang
*/
private void startLocation(){
// 设置定位参数
locationClient.setLocationOption(locationOption);
// 启动定位
locationClient.startLocation();
}
/**
* 默认的定位参数
* @since 2.8.0
* @author hongming.wang
*
*/
private AMapLocationClientOption getDefaultOption(){
AMapLocationClientOption mOption = new AMapLocationClientOption();
mOption.setLocationMode(AMapLocationClientOption.AMapLocationMode.Hight_Accuracy);//可选,设置定位模式,可选的模式有高精度、仅设备、仅网络。默认为高精度模式
mOption.setGpsFirst(false);//可选,设置是否gps优先,只在高精度模式下有效。默认关闭
mOption.setHttpTimeOut(30000);//可选,设置网络请求超时时间。默认为30秒。在仅设备模式下无效
mOption.setInterval(2000);//可选,设置定位间隔。默认为2秒
mOption.setNeedAddress(true);//可选,设置是否返回逆地理地址信息。默认是ture
mOption.setOnceLocation(true);//可选,设置是否单次定位。默认是false
mOption.setOnceLocationLatest(false);//可选,设置是否等待wifi刷新,默认为false.如果设置为true,会自动变为单次定位,持续定位时不要使用
AMapLocationClientOption.setLocationProtocol(AMapLocationClientOption.AMapLocationProtocol.HTTP);//可选, 设置网络请求的协议。可选HTTP或者HTTPS。默认为HTTP
return mOption;
}
/**
* 定位监听
*/
AMapLocationListener locationListener = new AMapLocationListener() {
@Override
public void onLocationChanged(AMapLocation loc) {
if (null != loc) {
//解析定位结果
String result = Utils.getLocationStr(loc);
/**
* sb.append("经 度 : " + location.getLongitude() + "\n");
sb.append("纬 度 : " + location.getLatitude() + "\n");
*/
if (Latitude == 0.0 && Longitude == 0.0) {
Longitude = loc.getLongitude();
Latitude = loc.getLatitude();
final String coutent = "教师已上传定位坐标,可以定位签到了";
startPositioning(coutent);
}else {
mLoading.dismiss();
double signLongitude = loc.getLongitude();
double signLatitude = loc.getLatitude();
distance = DistanceOfTwoPoints(Latitude,Longitude,signLatitude,signLongitude);
if (distance > 50)
{
mTimer.setOnTimeCompleteListener(new Anticlockwise.OnTimeCompleteListener()
{
@Override
public void onTimeComplete()
{
int num = 0;
startAttendance(num);
}
});
stopLocation();
}else {
mTimer.stop();
mTimer.initTime(10,0);
}
}
String ret = ""+Longitude + Latitude;
String jStr = Longitude+",";
String wStr = Latitude+"";
Log.d("定位结果:long = ",Longitude+"lat = "+Latitude);
// statuTV.setText(jStr+wStr);
// test3TV.setText("定位结果:long = "+Longitude+"lat = "+Latitude);
} else {
//statuTV.setText("定位失败,loc is null");
mLoading.dismiss();
}
}
};
/**
* 根据两点间经纬度坐标(double值),计算两点间距离,
* @param lat1
* @param lng1
* @param lat2
* @param lng2
* @return 距离:单位为米
*/
public static double DistanceOfTwoPoints(double lat1,double lng1,
double lat2,double lng2) {
double radLat1 = rad(lat1);
double radLat2 = rad(lat2);
double a = radLat1 - radLat2;
double b = rad(lng1) - rad(lng2);
double s = 2 * Math.asin(Math.sqrt(Math.pow(Math.sin(a / 2), 2)
+ Math.cos(radLat1) * Math.cos(radLat2)
* Math.pow(Math.sin(b / 2), 2)));
s = s * 6378137.0;
s = Math.round(s * 10000) / 10000;
return s;
}
//查询教师状态
private void signRequest(){
RequestBody requestBodyPost = new FormBody.Builder()
.add("sportsClassId",sportclassId)
.add("teacherId",teacherId)
.add("HaveAclassState","0")
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.TeacherCourseStart)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
JSONObject jsObj = new JSONObject(retStr);
String retStr = jsObj.getString("melodyClass");
if (retStr.equals("yes")) {
int num = 1;
startAttendance(num);
}
else if (retStr.equals("BeASubstituteInThe"))
{
//statuTV.setText("不是签到时间");
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("您的课程被代课中!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}else if (retStr.equals("NotClickOnTheClass"))
{
//statuTV.setText("服务器异常");
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("请至少一名学生签到成功后开启上课!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}else if (retStr.equals("Error"))
{
//statuTV.setText("服务器异常");
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("开启失败,数据异常1!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}else
{
//statuTV.setText("服务器异常");
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("开启失败,数据异常2!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}
} catch (JSONException e) {
e.printStackTrace();
//statuTV.setText("签到异常");
}
}
});
}
});
}
//开始上课录入接口
private void startAttendance( int number){
RequestBody requestBodyPost = new FormBody.Builder()
.add("teacherid",teacherId)
.add("HaveAclassState","0")
.add("attendState",number+"")
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.TeacherStartAttendance)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
JSONObject jsObj = new JSONObject(retStr);
if (jsObj.getString("melodyClass").equals("离职"))
{
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("上传考勤记录!") ;
builder.setPositiveButton("确定" , null );
builder.show();
}else
{
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("开始上课!") ;
builder.setPositiveButton("确定" , null );
builder.show();
}
} catch (JSONException e) {
e.printStackTrace();
//statuTV.setText("签到异常");
}
}
});
}
});
}
//开启或关闭签到通道
private void startAndClose(final String method)
{
RequestBody requestBodyPost = new FormBody.Builder()
.add("teacherid",teacherId)
.add("theKey","0")
.add("status",method)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.UpdateTheQrCodeKey)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
JSONObject jsObj = new JSONObject(retStr);
if (jsObj.getString("melodyClass").equals("yes"))
{
if (method.equals("1"))
{
initLocation();
startLocation();
mLoading.show();
layout.setVisibility(View.VISIBLE);
refresh_position.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
startLocation();
}
});
}else
{
layout.setVisibility(View.GONE);
if (Latitude == 0.0){
closeLocation();
}else {
stopLocation();
destroyLocation();
closeLocation();
}
}
}else
{
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("定位关闭失败") ;
builder.setPositiveButton("确定" , null );
builder.show();
}
} catch (JSONException e) {
e.printStackTrace();
//statuTV.setText("签到异常");
}
}
});
}
});
}
//存入教师坐标
private void startPositioning(final String content){
RequestBody requestBodyPost = new FormBody.Builder()
.add("teacherId",teacherId)
.add("longitude",Longitude+"")
.add("latitude",Latitude+"")
.add("classId",sportclassId)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.TeacherStartPositioning)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
Log.d("请求失败 teache",teacherId+"classId = "+sportclassId+"99999999999999999999999999999999999999999999");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
mLoading.dismiss();
try {
JSONObject jsObj = new JSONObject(retStr);
coordinateId = jsObj.getString("id");
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage(content) ;
String ret = ""+Longitude + Latitude;
Log.d("ret = ",ret);
builder.setPositiveButton("确定" , null );
builder.show();
} catch (JSONException e) {
e.printStackTrace();
//statuTV.setText("签到异常");
}
}
});
}
});
}
//关闭定位
private void closeLocation(){
RequestBody requestBodyPost = new FormBody.Builder()
.add("id",coordinateId)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.TeacherCloseLocation)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
JSONObject jsObj = new JSONObject(retStr);
if (jsObj.getString("Error").equals("yes"))
{
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("定位关闭成功!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}else
{
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("定位关闭失败") ;
builder.setPositiveButton("确定" , null );
builder.show();
}
} catch (JSONException e) {
e.printStackTrace();
//statuTV.setText("签到异常");
}
}
});
}
});
}
//下课请求
private void endOfCourse(){
RequestBody requestBodyPost = new FormBody.Builder()
.add("teacherId",teacherId)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.TeacherEndOfCourse)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
//signSleepTextView.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
JSONObject jsObj = new JSONObject(retStr);
datrr = new JSONObject(retStr);
classState = jsObj.getString("melodyClass");
if (classState.equals("yes")) {
//statuTV.setText("不是签到时间");
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("成功,本节课结束!" ) ;
builder.setPositiveButton("确定" , new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which) {
//进行页面跳转,跳回界面
Intent intent = new Intent(ClassListDetailsActivity.this, Class_ListActivity.class);
intent.putExtra("itemId",itemId);
intent.putExtra("teacherId",teacherId);
startActivity(intent);
finish();
}
});
builder.show();
}
else if (classState.equals("noTime"))
{
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("失败,上课时间不足,请稍后再试!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}else if (classState.equals("no"))
{
//statuTV.setText("服务器异常");
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("失败,未查询到上课!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}else if (classState.equals("Error"))
{
//statuTV.setText("服务器异常");
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("开启失败,数据异常1!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}else
{
//statuTV.setText("服务器异常");
AlertDialog.Builder builder = new AlertDialog.Builder(ClassListDetailsActivity.this);
builder.setTitle("提示" ) ;
builder.setMessage("开启失败,数据异常2!" ) ;
builder.setPositiveButton("确定" , null );
builder.show();
}
} catch (JSONException e) {
e.printStackTrace();
//statuTV.setText("签到异常");
}
}
});
}
});
}
/**
* 停止定位
*
* @since 2.8.0
* @author hongming.wang
*
*/
private void stopLocation(){
// 停止定位
locationClient.stopLocation();
}
@Override
protected void onDestroy() {
super.onDestroy();
destroyLocation();
}
/**
* 销毁定位
*
* @since 2.8.0
* @author hongming.wang
*
*/
private void destroyLocation(){
if (null != locationClient) {
/**
* 如果AMapLocationClient是在当前Activity实例化的,
* 在Activity的onDestroy中一定要执行AMapLocationClient的onDestroy
*/
locationClient.onDestroy();
locationClient = null;
locationOption = null;
}
}
private static double rad(double d) {
return d * Math.PI / 180.0;
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda.entity;
/**
* 管理员基类
*/
public class AdminModel {
/**
* birthday : null
* phone : 123456
* sex : 1
* status : 2
* jurisdiction : 1
* collegeName : null
* password : <PASSWORD>
* id : 67
* cardNo : null
* picture : null
* name : admin
* itro : null
* loginSta : success
*/
private String birthday;
private String phone;
private int sex;
private int status;
private String jurisdiction;
private String collegeName;
private String password;
private String id;
private String cardNo;
private String picture;
private String name;
private String itro;
private String loginSta;
public String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
this.birthday = birthday;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public int getSex() {
return sex;
}
public void setSex(int sex) {
this.sex = sex;
}
public int getStatus() {
return status;
}
public void setStatus(int status) {
this.status = status;
}
public String getJurisdiction() {
return jurisdiction;
}
public void setJurisdiction(String jurisdiction) {
this.jurisdiction = jurisdiction;
}
public String getCollegeName() {
return collegeName;
}
public void setCollegeName(String collegeName) {
this.collegeName = collegeName;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getCardNo() {
return cardNo;
}
public void setCardNo(String cardNo) {
this.cardNo = cardNo;
}
public String getPicture() {
return picture;
}
public void setPicture(String picture) {
this.picture = picture;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getItro() {
return itro;
}
public void setItro(String itro) {
this.itro = itro;
}
public String getLoginSta() {
return loginSta;
}
public void setLoginSta(String loginSta) {
this.loginSta = loginSta;
}
}
<file_sep>package com.xinnuo.apple.nongda.Binding;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import com.xinnuo.apple.nongda.MainActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.TeacherActivity.AccountSettings.AccountSettingsActivity;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class TeacherBindingActivity extends AppCompatActivity implements View.OnClickListener {
//定义控件
private EditText account;
private TextView tvshow;
private EditText pwd;
private Button login;
private static OkHttpClient client;
private static String melodyClass;
private String teacherId;
private String cardNo;
private String phone;
private String state;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_teacher_binding);
initOkHttp();
Intent intent = getIntent();
//取出登录界面传过来的教师id
cardNo = intent.getStringExtra("cardNo");
Log.d("cardNo = ", cardNo);
phone = intent.getStringExtra("phone");
teacherId = intent.getStringExtra("teacherId");
if (intent.getStringExtra("state") != null){
state = intent.getStringExtra("state");
}
//绑定控件
this.account = (EditText) findViewById(R.id.nameEditTV);
this.pwd = (EditText) findViewById(R.id.passwordEditTV);
this.login = (Button) findViewById(R.id.loginBtn);
this.tvshow = (TextView) findViewById(R.id.tv_show);
login.setOnClickListener(this);
}
// 实例化AlertDailog.Builder对象
public void onClick(View v) {
//接收页面传过来来的手机号和身份证号
final String userIdStr = account.getText().toString().trim();
final String passwordStr = pwd.getText().toString().trim();
// 设置显示信息
final AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage("确定要绑定?").
// 设置确定按钮
setPositiveButton("是",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which) {
// 设置TextView文本
//点击是的时候去进行提交
if (userIdStr.length() != 6 || passwordStr.length() != 11){
AlertDialog.Builder builder = new AlertDialog.Builder(TeacherBindingActivity.this);
builder.setTitle("提示");
builder.setMessage("手机号是11位,工号为6位!");
builder.setPositiveButton("确定", null);
builder.show();
}else{
requestWithUserId(userIdStr, passwordStr, teacherId);
}
}
}).
// 设置取消按钮
setNegativeButton("否",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
tvshow.setText("取消绑定!");
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
}
private void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
/**
* 请求方法 参数(工号、手机号、教师编号)
*/
private void requestWithUserId(String IdNumber, String Phone, String teacherid) {
RequestBody requestBodyPost = new FormBody.Builder()
.add("cardNo", IdNumber)
.add("phone", Phone)
.add("id", teacherid)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.teacherBindingUrl)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
tvshow.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收htttpresponse请求的返回值
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值", retStr);
try {
//调用解析方法 解析数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
e.printStackTrace();
}
}
});
}
});
}
//json数据解析
private void jsonParseWithJsonStr(String jsonStr) throws JSONException {
JSONArray jsArr = new JSONArray(jsonStr);
//绑定状态
String bindingState = null;
//循环jsArr集合
for (int i = 0; i < jsArr.length(); i++) {
JSONObject jsObj = jsArr.getJSONObject(i);
//取到state返回值
bindingState = jsObj.getString("state");
}
//判断bindingState
if (bindingState.equals("绑定成功")) {
tvshow.setText("绑定成功!");
//绑定成功后跳转登录界面
if (state.equals("1")){
Intent intent = new Intent(TeacherBindingActivity.this, MainActivity.class);
startActivity(intent);
finish();
}else {
Intent intent = new Intent(TeacherBindingActivity.this, AccountSettingsActivity.class);
intent.putExtra("phone",phone);
intent.putExtra("cardNo",cardNo);
intent.putExtra("teacherId",teacherId);
startActivity(intent);
finish();
}
} else {
//绑定失败留在本界面
tvshow.setText("绑定失败");
}
}
}
<file_sep>package com.xinnuo.apple.nongda.TeacherActivity.Resultquery;
/**
* 体质测试班级展示
* */
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class PhysicalFitnessTestActivity extends BaseActivity {
private OkHttpClient client;
private String classId;
private String sportsClassNo;
private ListView listView;
private String state;
private JSONArray dataArr;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_student_list_query);
initOkHttp();
listView = (ListView) findViewById(R.id.list_stuquerys);
Intent intent = getIntent();
sportsClassNo = intent.getStringExtra("sportsClassNo");
requestWithUserId(sportsClassNo, httpUrl.StudentPhysicalFitnessScore);
listView= (ListView)findViewById(R.id.list_stuquerys);
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
try {
//点击相应的班级进行跳转
Intent intent=new Intent(PhysicalFitnessTestActivity.this,StudentDetailedQueryActivity.class);
JSONObject js = dataArr.getJSONObject(position);
//传值 班级id 所教班级id
intent.putExtra("studentId",js.getString("studentId"));
startActivity(intent);
} catch (JSONException e) {
e.printStackTrace();
}
}
});
}
/**
* 请求方法
* */
private void requestWithUserId(String itemId,String url){
//菊花的开始方法
mLoading.show();
RequestBody requestBodyPost = new FormBody.Builder()
.add("itemId",itemId)
.build();
Request requestPost = new Request.Builder()
.url(url)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr,state);
} catch (JSONException e) {
mLoading.dismiss();
e.printStackTrace();
}
}
});
}
});
}
/**
* 解析数据方法
* */
//json解析方法
private void jsonParseWithJsonStr (String jsonStr , String state) throws JSONException {
JSONArray jsArr = new JSONArray(jsonStr);
dataArr = jsArr;
List<String> data = new ArrayList<String>();
ArrayList<HashMap<String, Object>> listItem = new ArrayList<HashMap<String, Object>>();/*在数组中存放数据*/
HashMap<String, Object> map2 = new HashMap<String, Object>();
map2.put("count", "序 号");
map2.put("studentNo", " 学 号");
map2.put("stuname", "姓 名");
map2.put("number", "总 分");
listItem.add(map2);
for (int i = 0; i < jsArr.length(); i++) {
JSONObject js = jsArr.getJSONObject(i);
/*
* "studentNo": "11042227 ",
* "name": "张烁",
* "number": 0
*/
String count = i + 1 + "";
String studentNo = js.optString("studentId");
String stuname = js.optString("name");
String number = js.optString("result");
Log.d("******", studentNo);
HashMap<String, Object> map = new HashMap<String, Object>();
// map.put("id",id);
map.put("count", count);
map.put("studentNo", studentNo);
map.put("stuname", stuname);
map.put("number", number);
listItem.add(map);
}
if (jsArr.length() == 0)
{
midToast("无数据",3);
}
SimpleAdapter qrAdapter = new SimpleAdapter(this,listItem, R.layout.item_student_list_query,
new String[]{"count","studentNo","stuname","number"},
new int[]{R.id.studentlist_query1, R.id.studentlist_query2, R.id.studentlist_query3, R.id.studentlist_query4});
listView.setAdapter(qrAdapter);
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda.TeacherActivity.WorkPlan;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.TextView;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class TeacherAddWorkPlan extends BaseActivity {
private EditText title1;
private EditText createDate1;
private EditText beginDate1;
private EditText endDate1;
private EditText workContent1;
private EditText summary1;
private CheckBox work_yes;
private CheckBox work_no;
private TextView workplan_submit;
private OkHttpClient client;
private String title;
private String createDate;
private String beginDate;
private String endDate;
private String workContent;
private String state;
private String summary;
private String teacherId;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_teacher_add_work_plan);
initOkHttp();
binding();
SimpleDateFormat formatter = new SimpleDateFormat ("yyyy-MM-dd");
Date curDate = new Date(System.currentTimeMillis());//获取当前时间
createDate = formatter.format(curDate);
Intent intent = getIntent();
teacherId = intent.getStringExtra("teacherId");
state = "0";
createDate1.setText(createDate);
workplan_submit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
title = title1.getText().toString();
beginDate = beginDate1.getText().toString();
endDate = endDate1.getText().toString();
workContent = workContent1.getText().toString();
summary = summary1.getText().toString();
work_no.setChecked(true);
Log.d("dawdaw","+++"+title+"++++++++++++++++++++++++++++");
if (title.length() == 0){
AlertDialog.Builder builder = new AlertDialog.Builder(TeacherAddWorkPlan.this);
builder.setTitle("提示");
builder.setMessage("标题不能为空!");
builder.setPositiveButton("确定", null);
builder.show();
}else if (beginDate.length() == 0){
AlertDialog.Builder builder = new AlertDialog.Builder(TeacherAddWorkPlan.this);
builder.setTitle("提示");
builder.setMessage("开始时间不能为空!");
builder.setPositiveButton("确定", null);
builder.show();
}else if (endDate.length() == 0){
AlertDialog.Builder builder = new AlertDialog.Builder(TeacherAddWorkPlan.this);
builder.setTitle("提示");
builder.setMessage("结束时间不能为空!");
builder.setPositiveButton("确定", null);
builder.show();
}else if (workContent.length() == 0){
AlertDialog.Builder builder = new AlertDialog.Builder(TeacherAddWorkPlan.this);
builder.setTitle("提示");
builder.setMessage("计划不能为空!");
builder.setPositiveButton("确定", null);
builder.show();
}else if (summary.length() == 0){
AlertDialog.Builder builder = new AlertDialog.Builder(TeacherAddWorkPlan.this);
builder.setTitle("提示");
builder.setMessage("总结不能为空!");
builder.setPositiveButton("确定", null);
builder.show();
}else{
// 设置显示信息
final AlertDialog.Builder builder = new AlertDialog.Builder(TeacherAddWorkPlan.this);
builder.setMessage("是否提交").
// 设置确定按钮
setPositiveButton("提交",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which)
{
// 设置TextView文本
//点击是的时候去进行提交
// 单击事件
requestWithUserId();
}
}).
// 设置取消按钮
setNegativeButton("取消",
new DialogInterface.OnClickListener()
{
public void onClick(DialogInterface dialog,
int which)
{
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
builder.show();
}
}
});
}
//绑定控件
private void binding()
{
title1 = (EditText) findViewById(R.id.add_work_title);
createDate1 = (EditText) findViewById(R.id.add_work_createdate);
beginDate1 = (EditText) findViewById(R.id.add_work_startdate);
endDate1 = (EditText) findViewById(R.id.add_work_enddate);
workContent1 = (EditText) findViewById(R.id.add_work_plan);
summary1 = (EditText) findViewById(R.id.add_work_summary);
work_no = (CheckBox) findViewById(R.id.add_workplan_no);
work_yes = (CheckBox) findViewById(R.id.add_workplan_yes);
workplan_submit = (TextView) findViewById(R.id.workplan_submit);
}
/**
* 请求方法
* */
private void requestWithUserId(){
//菊花的开始方法
mLoading.show();
RequestBody requestBodyPost = new FormBody.Builder()
.add("title",title)
.add("createDate",createDate)
.add("beginDate",beginDate)
.add("endDate",endDate)
.add("workContent",workContent)
.add("state",state)
.add("summary",summary)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.AddWorkPlan)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
mLoading.dismiss();
e.printStackTrace();
}
}
});
}
});
}
/**
* 解析数据方法
* */
private void jsonParseWithJsonStr (String jsonStr) throws JSONException {
JSONArray jaArr = new JSONArray(jsonStr);
for (int i = 0 ; i < jaArr.length() ; i++){
JSONObject json = jaArr.getJSONObject(i);
String updateYesOrNo = json.getString("state");
if (updateYesOrNo.equals("增加成功"))
{
AlertDialog.Builder builder = new AlertDialog.Builder(TeacherAddWorkPlan.this);
builder.setTitle("提示");
builder.setMessage("增加成功!");
builder.setPositiveButton("确定",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which) {
// 设置TextView文本
//点击是的时候去进行提交
Intent intent = new Intent(TeacherAddWorkPlan.this,TeacherWorkPlan.class);
intent.putExtra("teacherId",teacherId);
startActivity(intent);
finish();
}
});
builder.show();
}else
{
AlertDialog.Builder builder = new AlertDialog.Builder(TeacherAddWorkPlan.this);
builder.setTitle("提示");
builder.setMessage("增加失败!");
builder.setPositiveButton("确定", null);
builder.show();
}
}
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda;
/**
* 电信号段正则表达式 根据传过来的电话号判断是否是电信号段
* */
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Created by Administrator on 2016/11/1 0001.
*/
public class IsPhoneNumUtil {
public static boolean isMobileNumber(String phoneNumber) {
Pattern pattern = Pattern.compile("^(133|149|153|173|177|180|181|189)\\d{8}$");
Matcher matcher = pattern.matcher(phoneNumber);
return matcher.matches();
}
}
<file_sep>package com.xinnuo.apple.nongda.studentActivity.TeacherEvaluation;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.LinearLayout;
import android.widget.TextView;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class TeacherEvaluationActivity extends BaseActivity {
private String id;
private TextView teacherName;
private TextView teacherState;
private LinearLayout teacherLayout;
private OkHttpClient client;
private String type = null,teacherId;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_teacher_evaluation);
initOkHttp();
final Intent intent = getIntent();
id = intent.getStringExtra("id");
teacherName = (TextView) findViewById(R.id.teacher_name);
teacherState = (TextView) findViewById(R.id.teacher_state);
teacherLayout = (LinearLayout) findViewById(R.id.teacher_Layout);
requestWithUserId();
teacherLayout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (type.equals("未评价")){
Intent intent1 = new Intent(TeacherEvaluationActivity.this,TeacherScoreActivity.class);
intent1.putExtra("teacherId",teacherId);
intent1.putExtra("id",id);
startActivity(intent1);
}
}
});
}
/**
* 请求方法
* */
private void requestWithUserId(){
//菊花的开始方法
mLoading.show();
RequestBody requestBodyPost = new FormBody.Builder()
.add("id",id)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.TeacherEvaluation)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
//接收方法
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse返回的json数据
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
//菊花结束方法
mLoading.dismiss();
try {
//解析json数据
jsonParseWithJsonStr(retStr);
} catch (Exception e) {
mLoading.dismiss();
e.printStackTrace();
}
}
});
}
});
}
/**
* 解析数据方法
* */
private void jsonParseWithJsonStr (String jsonStr){
JSONArray jsArr = null;
try {
jsArr = new JSONArray(jsonStr);
List<String> data = new ArrayList<String>();
ArrayList<HashMap<String, Object>> listItem = new ArrayList<HashMap<String, Object>>();/*在数组中存放数据*/
for (int i = 0; i < jsArr.length(); i++)
{
JSONObject js = jsArr.getJSONObject(i);
String meetType;
if (js.getString("type").equals("nul"))
{
type = "未评价";
teacherState.setText("未评价");
}else
{
type = "已评价";
teacherState.setText("已评价");
}
teacherName.setText(js.getString("name"));
teacherId = js.getString("id");
}
if (jsArr.length() == 0)
{
midToast("无数据",3);
}
} catch (JSONException e) {
e.printStackTrace();
AlertDialog.Builder builder = new AlertDialog.Builder(TeacherEvaluationActivity.this);
builder.setTitle("提示");
builder.setMessage("请先选择课程!");
builder.setPositiveButton("确定", new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which)
{
// 设置TextView文本
//点击是的时候去进行提交
finish();
}
});
builder.show();
}
}
/**
* 初始化网络请求
* */
public void initOkHttp() {
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
}
<file_sep>package com.xinnuo.apple.nongda.singin;
import android.app.Activity;
import android.content.Intent;
import android.util.Log;
import com.xinnuo.apple.nongda.BaseActivity;
import com.xinnuo.apple.nongda.TeacherActivity.TeacherActivity;
import com.xinnuo.apple.nongda.entity.TeacherModel;
import org.json.JSONException;
import org.json.JSONObject;
/**
* Created by Administrator on 2016/11/10 0010.
*/
public class Teacher_Singin extends BaseActivity {
public Intent teacher_Login(Activity activity, JSONObject jsObj , TeacherModel teacherInfo)
{
Intent intent = null;
try {
setTeacherInfo(jsObj);
//跳转界面并进行传值 教师姓名 教的班级 教师姓名
intent = new Intent(activity, TeacherActivity.class);
intent.putExtra("teacherId", teacherInfo.getId());
intent.putExtra("itemId", teacherInfo.getItemId());
intent.putExtra("teacherName",teacherInfo.getName());
Log.d("*****",teacherInfo.getName());
} catch (JSONException e) {
e.printStackTrace();
}
return intent;
}
//教师model
public TeacherModel setTeacherInfo(JSONObject jsObj) throws JSONException {
TeacherModel teacher = new TeacherModel();
teacher.setBirthday(jsObj.getString("birthday"));
teacher.setPhone(jsObj.getString("phone"));
teacher.setSex(jsObj.getInt("sex"));
teacher.setStatus(jsObj.getInt("status"));
teacher.setJurisdiction(jsObj.getString("jurisdiction"));
teacher.setItemId(jsObj.getString("itemId"));
teacher.setCollegeName(jsObj.getString("collegeName"));
teacher.setPassword(jsObj.getString("password"));
teacher.setIntro(jsObj.getString("intro"));
teacher.setId(jsObj.getString("id"));
teacher.setCardNo(jsObj.getString("cardNo"));
teacher.setPicture(jsObj.getString("picture"));
teacher.setNationName(jsObj.getString("nationName"));
teacher.setName(jsObj.getString("name"));
teacher.setLoginSta(jsObj.getString("loginSta"));
return teacher;
}
}
<file_sep>package com.xinnuo.apple.nongda.Binding;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import com.xinnuo.apple.nongda.MainActivity;
import com.xinnuo.apple.nongda.R;
import com.xinnuo.apple.nongda.httpUrl;
import com.xinnuo.apple.nongda.studentActivity.AccountSettings.StuAccountSettingsActivity;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
public class StudentBindingActivity extends AppCompatActivity implements View.OnClickListener {
//定义控件
private EditText account;
private TextView tvshow;
private EditText pwd;
private Button login;
private static OkHttpClient client;
private static String melodyClass;
private static String userNo;
private String id;
private String studentNo;
private String password;
private String new_paswd;
private String state;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_student_binding);
initOkHttp();
Intent intent = getIntent();
id = intent.getStringExtra("id");
studentNo = intent.getStringExtra("studentNo");
Log.d("stuNo = ",studentNo);
password = intent.getStringExtra("password");
if (intent.getStringExtra("state") != null){
state = intent.getStringExtra("state");
}
initOkHttp();
//绑定控件
this.account = (EditText) findViewById(R.id.nameEditTV);
this.pwd = (EditText) findViewById(R.id.passwordEditTV);
this.login = (Button) findViewById(R.id.loginBtn);
this.tvshow = (TextView) findViewById(R.id.tv_show);
login.setOnClickListener(this);
}
// 实例化AlertDailog.Builder对象
@Override
public void onClick(View v) {
//接收页面传过来来的手机号和身份证号
final String userIdStr = account.getText().toString().trim();
final String passwordStr = pwd.getText().toString().trim();
Log.d("userIdStr",userIdStr);
Log.d("passwordStr",passwordStr);
// 设置显示信息
final AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage("确定要绑定?").
// 设置确定按钮
setPositiveButton("是",
new DialogInterface.OnClickListener() {
// 单击事件
public void onClick(DialogInterface dialog, int which) {
// 设置TextView文本
//点击是的时候去进行提交
if (userIdStr.length() != 18 || passwordStr.length() != 11){
AlertDialog.Builder builder = new AlertDialog.Builder(StudentBindingActivity.this);
builder.setTitle("提示");
builder.setMessage("手机号是11位,身份证号是18位!");
builder.setPositiveButton("确定", null);
builder.show();
} else {
requestWithUserId(userIdStr,passwordStr,studentNo);
}
}
}).
// 设置取消按钮
setNegativeButton("否",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int which) {
tvshow.setText("取消绑定!");
}
});
// 创建对话框
AlertDialog ad = builder.create();
// 显示对话框
ad.show();
}
private void initOkHttp()
{
client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build();
}
/**
* 请求方法 参数(身份证号、电话号、学生编号)
* */
private void requestWithUserId(String IdNumber , String Phone , String StudentNo){
RequestBody requestBodyPost = new FormBody.Builder()
.add("IdNumber",IdNumber)
.add("Phone",Phone)
.add("stuNo",StudentNo)
.build();
Request requestPost = new Request.Builder()
.url(httpUrl.studentBindingUrl)
.post(requestBodyPost)
.build();
client.newCall(requestPost).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
tvshow.setText("网络异常!");
}
@Override
public void onResponse(Call call, Response response) throws IOException {
//接收httpresponse请求的返回值
final String retStr = response.body().string();
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d("网络请求返回值",retStr);
try {
//调用解析方法 解析数据
jsonParseWithJsonStr(retStr);
} catch (JSONException e) {
e.printStackTrace();
}
}
});
}
});
}
//json数据解析
private void jsonParseWithJsonStr (String jsonStr) throws JSONException {
JSONObject js = new JSONObject(jsonStr);
melodyClass = js.getString("melodyClass");
Log.d("*******************",melodyClass);
//判断返回值如果是yes就跳转。如果是no就给提示信息
if (melodyClass.equals("yes")){
tvshow.setText("绑定成功!");
//绑定成功后跳转登录界面
if (state.equals("1")){
Intent intent = new Intent(StudentBindingActivity.this,MainActivity.class);
startActivity(intent);
finish();
}else{
Intent intent = new Intent(StudentBindingActivity.this,StuAccountSettingsActivity.class);
intent.putExtra("id",id);
intent.putExtra("studentNo",studentNo);
intent.putExtra("password",<PASSWORD>);
startActivity(intent);
//清理缓存
finish();
}
}else{
//绑定失败留在本界面
tvshow.setText("绑定失败");
}
}
}
<file_sep>package com.xinnuo.apple.nongda.entity;
/**
* 学生基类
*/
public class StudentModel {
/**
* cardId : 11042227
* birthday : 1993/7/10
* phone : 18603603980
* studentNo : 11042227
* sex : 2
* gradeId : 3
* status : 1
* classId : 5394
* electiveInformation : null
* password : <PASSWORD>
* collegeName : 工学院
* nationId : 汉族
* id : 37248
* stuSource : null
* homeAddress : null
* name : 张烁
* className : 食品13-2
* loginSta : successToStu
* reelect : 1
* idNumber : 230107199505122016
*/
private String cardId;
private String birthday;
private String phone;
private String studentNo;
private String sex;
private int gradeId;
private int status;
private String classId;
private String electiveInformation;
private String password;
private String collegeName;
private String nationId;
private String id;
private String stuSource;
private String homeAddress;
private String name;
private String className;
private String loginSta;
private int reelect;
private String idNumber;
public String getCardId() {
return cardId;
}
public void setCardId(String cardId) {
this.cardId = cardId;
}
public String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
this.birthday = birthday;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getStudentNo() {
return studentNo;
}
public void setStudentNo(String studentNo) {
this.studentNo = studentNo;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public int getGradeId() {
return gradeId;
}
public void setGradeId(int gradeId) {
this.gradeId = gradeId;
}
public int getStatus() {
return status;
}
public void setStatus(int status) {
this.status = status;
}
public String getClassId() {
return classId;
}
public void setClassId(String classId) {
this.classId = classId;
}
public String getElectiveInformation() {
return electiveInformation;
}
public void setElectiveInformation(String electiveInformation) {
this.electiveInformation = electiveInformation;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = <PASSWORD>;
}
public String getCollegeName() {
return collegeName;
}
public void setCollegeName(String collegeName) {
this.collegeName = collegeName;
}
public String getNationId() {
return nationId;
}
public void setNationId(String nationId) {
this.nationId = nationId;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getStuSource() {
return stuSource;
}
public void setStuSource(String stuSource) {
this.stuSource = stuSource;
}
public String getHomeAddress() {
return homeAddress;
}
public void setHomeAddress(String homeAddress) {
this.homeAddress = homeAddress;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getClassName() {
return className;
}
public void setClassName(String className) {
this.className = className;
}
public String getLoginSta() {
return loginSta;
}
public void setLoginSta(String loginSta) {
this.loginSta = loginSta;
}
public int getReelect() {
return reelect;
}
public void setReelect(int reelect) {
this.reelect = reelect;
}
public String getIdNumber() {
return idNumber;
}
public void setIdNumber(String idNumber) {
this.idNumber = idNumber;
}
}
| 1ba5523cec9848237f8adea7a4dde51038c7ce73 | [
"Java"
] | 35 | Java | cong1300/nongda | dabad7367f1a86854171edbc1d74a6fabcb5e3bd | 5b80a4d48af6c993a6d5767f56b85831e8c7c6d8 | |
refs/heads/master | <file_sep>#ifndef WEATHERWIDGET_H
#define WEATHERWIDGET_H
#include <QWidget>
#include <QUrl>
#include "downloader.h"
#include <QProgressBar>
#include <QLineEdit>
#include <QPushButton>
#include <QByteArray>
#include <QTextEdit>
#include <QString>
#include "picojson.h"
#include <QMap>
#include "logger.h"
namespace Ui {
class Form;
}
class WeatherWidget : public QWidget
{
Q_OBJECT
private:
Ui::Form* ui;
Downloader* pdl_;
Downloader* pdlImg_;
QString urlRoot_;
QString weatherQuery_;
QString weatherImgQuery_;
QString imgExtention_;
const QString APPID_;
typedef QMap<QString, QByteArray> ImgCache;
ImgCache cache_;
private:
void JSONParseAndFill(picojson::value::object& obj);
void JSONParseAndFill(picojson::value::array& obj);
void displayError(const QString& title, const QString& message);
void setText(const QString& widgetName, const QString& value);
void downloadImg(const QString& imgName);
const QByteArray* checkCache(const QString& key);
public:
explicit WeatherWidget(QWidget *parent = 0);
~WeatherWidget();
void connectLogger(const Logger& logger);
public slots:
void slotGo (const QString&);
void slotClearFroms ();
private slots:
void slotError ();
void slotDone (const QUrl&, const QByteArray&);
void slotImgDone (const QUrl&, const QByteArray&);
void slotResetToDefault (bool);
void slotError (QString errStatus);
};
#endif // WEATHERWIDGET_H
<file_sep>#ifndef DOWNLOADER_H
#define DOWNLOADER_H
#include <QObject>
#include <QNetworkAccessManager>
#include <QNetworkReply>
class Downloader : public QObject
{
Q_OBJECT
private:
QNetworkAccessManager* pnam_;
QString getStringError(QNetworkReply::NetworkError);
public:
explicit Downloader(QObject *parent = 0);
void download(const QUrl&);
signals:
void done(const QUrl&, const QByteArray&);
void error(const QString);
private slots:
void slotFinished(QNetworkReply*);
};
#endif // DOWNLOADER_H
<file_sep>#include "downloader.h"
#include <QNetworkRequest>
QString Downloader::getStringError(QNetworkReply::NetworkError err)
{
QString error;
switch (err) {
case QNetworkReply::ConnectionRefusedError: error = "the remote server refused the connection (the server is not accepting requests)"; break;
case QNetworkReply::RemoteHostClosedError: error = "the remote server closed the connection prematurely, before the entire reply was received and processed"; break;
case QNetworkReply::HostNotFoundError: error = "the remote host name was not found (invalid hostname)"; break;
case QNetworkReply::TimeoutError: error = "the connection to the remote server timed out"; break;
case QNetworkReply::OperationCanceledError: error = "the operation was canceled via calls to abort() or close() before it was finished"; break;
case QNetworkReply::SslHandshakeFailedError: error = "the SSL/TLS handshake failed and the encrypted channel could not be established. The sslErrors() signal should have been emitted"; break;
case QNetworkReply::TemporaryNetworkFailureError: error = "the connection was broken due to disconnection from the network, however the system has initiated roaming to another access point. The request should be resubmitted and will be processed as soon as the connection is re-established."; break;
case QNetworkReply::ProxyConnectionRefusedError: error = "the connection to the proxy server was refused (the proxy server is not accepting requests)"; break;
case QNetworkReply::ProxyConnectionClosedError: error = "the proxy server closed the connection prematurely, before the entire reply was received and processed"; break;
case QNetworkReply::ProxyNotFoundError: error = "the proxy host name was not found (invalid proxy hostname)"; break;
case QNetworkReply::ProxyTimeoutError: error = "the connection to the proxy timed out or the proxy did not reply in time to the request sent"; break;
case QNetworkReply::ProxyAuthenticationRequiredError: error = "the proxy requires authentication in order to honour the request but did not accept any credentials offered (if any)"; break;
case QNetworkReply::ContentAccessDenied: error = "the access to the remote content was denied (similar to HTTP error 401)"; break;
case QNetworkReply::ContentOperationNotPermittedError: error = "the operation requested on the remote content is not permitted"; break;
case QNetworkReply::ContentNotFoundError: error = "the remote content was not found at the server (similar to HTTP error 404)"; break;
case QNetworkReply::AuthenticationRequiredError: error = "the remote server requires authentication to serve the content but the credentials provided were not accepted (if any)"; break;
case QNetworkReply::ContentReSendError: error = "the request needed to be sent again, but this failed for example because the upload data could not be read a second time."; break;
case QNetworkReply::ProtocolUnknownError: error = "the Network Access API cannot honor the request because the protocol is not known"; break;
case QNetworkReply::ProtocolInvalidOperationError: error = "the requested operation is invalid for this protocol"; break;
case QNetworkReply::UnknownNetworkError: error = "an unknown network-related error was detected"; break;
case QNetworkReply::UnknownProxyError: error = "an unknown proxy-related error was detected"; break;
case QNetworkReply::UnknownContentError: error = "an unknown error related to the remote content was detected"; break;
case QNetworkReply::ProtocolFailure: error = "a breakdown in protocol was detected (parsing error, invalid or unexpected responses, etc.)"; break;
default:
error = "unkown error occured";
break;
}
return error;
}
Downloader::Downloader(QObject *parent) :
QObject(parent)
{
pnam_ = new QNetworkAccessManager(this);
connect(pnam_, SIGNAL(finished(QNetworkReply*)),
this, SLOT(slotFinished(QNetworkReply*))
);
}
void Downloader::download(const QUrl & url)
{
QNetworkRequest request(url);
QNetworkReply* reply = pnam_->get(request);
}
void Downloader::slotFinished(QNetworkReply *reply)
{
if(reply->error() != QNetworkReply::NoError){
emit error(getStringError(reply->error()));
} else {
emit done(reply->url(), reply->readAll());
}
reply->deleteLater();
}
<file_sep>#ifndef DATA_H
#define DATA_H
#include <memory>
#include <QString>
#include <QByteArray>
#include <iostream>
class City;
class Coord
{
friend class City;
public:
Coord(double lon, double lat) : lon_(lon), lat_(lat){}
double lon() const;
double lat() const;
private:
double lon_;
double lat_;
Coord() : lon_(0), lat_(0){}
};
class City {
public:
static std::shared_ptr<City> makeCity(const QByteArray& JSONRaw);
City(unsigned int id, const QString& name, const QString& country, const Coord& coord);
unsigned id() const;
const QString& name() const;
const QString& country() const;
const Coord& coord() const;
private:
unsigned int id_;
QString name_;
QString country_;
Coord coord_;
static City* hardCodeParse(const QByteArray& JSONRaw);
City();
};
class Data
{
public:
Data();
};
#endif // DATA_H
std::ostream &operator<<(std::ostream& out, const City& city);
<file_sep>#include "data.h"
#include <iomanip>
#include <QDebug>
#include <QByteArray>
#include <cassert>
Data::Data()
{
}
double Coord::lon() const
{
return lon_;
}
double Coord::lat() const
{
return lat_;
}
std::shared_ptr<City> City::makeCity(const QByteArray& JSONRaw)
{
City* p = hardCodeParse(JSONRaw);
if (!p) {
return std::shared_ptr<City>();
}
return std::shared_ptr<City>(p);
}
City::City(unsigned int id, const QString& name, const QString& country, const Coord& coord) :
id_(id),
name_(name),
country_(country),
coord_(coord)
{
}
unsigned City::id() const
{
return id_;
}
const QString &City::name() const
{
return name_;
}
const QString &City::country() const
{
return country_;
}
const Coord &City::coord() const
{
return coord_;
}
City *City::hardCodeParse(const QByteArray& JSONRaw)
{ //TODO negative lon lat to implement
City* newCity = new City;
newCity->id_= 0;
const char* curr = JSONRaw.data();
unsigned char divide_counter = 0;
static double pow_10[] = { 10.0, 100.0, 1000.0, 10000.0, 100000.0, 1000000.0, 10000000.0, 100000000.0, 1000000000.0 };
QByteArray cityName;
QByteArray countryName;
enum STATES {
start,
first_colon,
first_colon_comma,
second_colon,
second_colon_open_quote,
second_colon_close_quote,
third_colon,
third_colon_open_quote,
third_colon_close_quote,
forth_colon,
fifth_colon,
fifth_colon_dot,
fifth_colon_comma,
sixth_colon,
sixth_colon_dot,
sixth_colon_right_bracket,
enough
};
STATES state = start;
while (*curr) {
char ch = *curr;
switch (state) {
case start:
if ( ch == ':' ) {
state = first_colon;
}
break;
case first_colon:
if ( ch == ',' ) {
state = first_colon_comma;
} else {
if ( ch >= '0' && ch <= '9' ){
newCity->id_ = newCity->id_ *10 +(ch - '0');
} else {
return nullptr;
}
}
break;
case first_colon_comma:
if ( ch == ':' ) {
state = second_colon;
}
break;
case second_colon:
if ( ch == '\"' ) {
state = second_colon_open_quote;
}
break;
case second_colon_open_quote:
if ( ch == '\"' ){
state = second_colon_close_quote;
} else {
cityName.append(ch);
}
break;
case second_colon_close_quote:
if ( ch == ':' ) {
state = third_colon;
}
break;
case third_colon:
if ( ch == '\"' ) {
state = third_colon_open_quote;
}
break;
case third_colon_open_quote:
if ( ch == '\"' ){
state = third_colon_close_quote;
} else {
countryName.append(ch);
}
break;
case third_colon_close_quote:
if ( ch == ':' ) {
state = forth_colon;
}
break;
case forth_colon:
if ( ch == ':' ) {
state = fifth_colon;
}
break;
case fifth_colon:
if ( ch == '.' ) {
state = fifth_colon_dot;
} else if ( ch >= '0' && ch <= '9' ) {
newCity->coord_.lon_ = newCity->coord_.lon_*10 +(ch - '0');
} else {
return nullptr;
}
break;
case fifth_colon_dot:
if ( ch == ',' ) {
state = fifth_colon_comma;
divide_counter = 0;
} else if ( ch >= '0' && ch <= '9' ) {
newCity->coord_.lon_ += (ch - '0') / pow_10[divide_counter++];
} else {
return nullptr;
}
break;
case fifth_colon_comma:
if ( ch == ':' ) {
state = sixth_colon;
}
break;
case sixth_colon:
if ( ch == '.' ) {
state = sixth_colon_dot;
} else if ( ch >= '0' && ch <= '9' ) {
newCity->coord_.lat_ = newCity->coord_.lat_*10 +(ch - '0');
} else {
return nullptr;
}
break;
case sixth_colon_dot:
if ( ch == '}' ) {
state = sixth_colon_right_bracket;
} else if ( ch >= '0' && ch <= '9' ) {
newCity->coord_.lat_ += (ch - '0') / pow_10[divide_counter++];
} else {
return nullptr;
}
break;
case sixth_colon_right_bracket:
state = enough;
break;
case enough:
assert("We can't be here");
break;
};
if ( state == enough ) {
break; // break while
}
++curr;
}
newCity->name_.append(cityName);
newCity->country_.append(countryName);
return newCity;
}
City::City()
{
}
std::ostream& operator<<(std::ostream &out, const City &city)
{
qDebug() << "_________Start__________\n";
qDebug() << "Name: " << city.name();// << std::endl;
qDebug() << "id: " << city.id();
qDebug() << "country: " << city.country();
qDebug() << "coord.lon: " << city.coord().lon();
qDebug() << "coord.lat: " << city.coord().lat();
qDebug() << "_________Finish__________\n";
return out;
}
<file_sep>#include "logger.h"
#include <QFileDialog>
#include <QDebug>
#include <QDir>
#include <QMessageBox>
#include <cassert>
#include <QDateTime>
Logger::Logger( mode::ENUM m, QObject* parrent ) :
QObject(parrent)
{
assert( (m == mode::append || m == mode::clear) && "Unknown mode");
QString currDir = QDir::currentPath();
bool isFileSelected = false;
while ( !isFileSelected ) {
do {
logFileName_ = QFileDialog::getOpenFileName(0, "Select the log file", currDir);
}
while( logFileName_.isEmpty() );
log_.setFileName(logFileName_);
QIODevice::OpenMode openMode;
openMode = (m == mode::append) ? QIODevice::Append : QIODevice::Truncate;
log_.open(QIODevice::WriteOnly|openMode);
if ( !log_.isWritable() ) {
QMessageBox::critical(0, "Error",
"Can not write to the file, please chose another file"
);
log_.close();
} else {
isFileSelected = true;
}
} //end while (!isFileSelected)
markStartMessage();
}
Logger::~Logger()
{
markEndMessage();
}
Logger& Logger::operator<<(const QString &logThis)
{
QDateTime now = QDateTime::currentDateTime();
QString date = now.toString() + " ";
//qDebug() << date;
log_.write(date.toStdString().c_str(), date.toStdString().size());
log_.write(logThis.toStdString().c_str(), logThis.toStdString().size());
addNextLine();
return *this;
}
void Logger::markStartMessage()
{
QString message = " Writing started";
(*this) << message;
addNextLine();
}
void Logger::markEndMessage()
{
QString message = " Writing finished";
(*this) << message;
addNextLine();
log_.flush();
}
void Logger::addNextLine()
{
#if defined(Q_OS_WIN) || defined(Q_OS_WIN32) || defined(Q_OS_OS2)
log_.write("\r\n", 2);
#elif defined Q_OS_MAC
log_.write("\n", 1);
#endif
}
void Logger::slotLog(const QUrl& url,const QByteArray& ba)
{
slotLog(url);
(*this) << QString(ba);
}
void Logger::slotLog(const QUrl& url)
{
(*this) << url.toString();
}
<file_sep>#ifndef LO_TREEWIDGET_H
#define LO_TREEWIDGET_H
#include <QTreeWidget>
#include <QObject>
#include <QWidget>
#include <QHash>
class LO_TreeWidget : public QTreeWidget
{
Q_OBJECT
private:
QString sourceFileName_;
public:
explicit LO_TreeWidget(const QString& sourceFileName, QWidget *parent = 0);
bool loadData();
signals:
void activated(const QString& cityName);
protected slots:
void slot_emitActivated(QTreeWidgetItem*,int);
};
#endif // LO_TREEWIDGET_H
<file_sep>#include "weatherwidget.h"
#include <QGridLayout>
#include <QDebug>
#include <QMessageBox>
#include <iostream>
#include "ui_weather_display.h"
#include <QWidget>
#include <QList>
WeatherWidget::WeatherWidget(QWidget *parent) :
QWidget(parent),
ui(new Ui::Form),
APPID_("<KEY>")
{
ui->setupUi(this);
urlRoot_ = "http://api.openweathermap.org/";
weatherQuery_ = "data/2.5/weather/?q=";
weatherImgQuery_ = "img/w/";
imgExtention_ = ".png";
ui->APPID->setText(APPID_);
pdl_ = new Downloader(this);
pdlImg_ = new Downloader(this);
connect(pdl_, SIGNAL(done(const QUrl&,const QByteArray&)), this, SLOT(slotDone(const QUrl&,const QByteArray&)));
connect(pdlImg_, SIGNAL(done(const QUrl&,const QByteArray&)), this, SLOT(slotImgDone(const QUrl&,const QByteArray&)));
connect(ui->btn_resetAPPID, SIGNAL(clicked(bool)), this, SLOT(slotResetToDefault(bool)));
connect(pdl_, SIGNAL(error(QString)), this, SLOT(slotError(QString)));
}
WeatherWidget::~WeatherWidget()
{
delete ui;
}
void WeatherWidget::connectLogger(const Logger &logger)
{
connect(pdl_, SIGNAL(done(const QUrl&,const QByteArray&)),
&logger, SLOT(slotLog(const QUrl&,const QByteArray&))
);
connect(pdlImg_, SIGNAL(done(const QUrl&,const QByteArray&)),
&logger, SLOT(slotLog(const QUrl&))
);
}
void WeatherWidget::slotGo(const QString & url)
{
slotClearFroms();
QString request = urlRoot_ + weatherQuery_ + url +"&APPID=" + ui->APPID->text();
pdl_->download(QUrl(request));
}
void WeatherWidget::slotClearFroms()
{
{
QList<QLineEdit*> list = ui->layoutWidget->findChildren<QLineEdit*>();
QList<QLineEdit*>::iterator iter = list.begin();
for( ; iter != list.end(); ++iter ) {
if ( (*iter)->objectName() == QString("APPID") ){
continue;
}
(*iter)->clear();
}
}
{
QList<QTextEdit*> list = ui->layoutWidget->findChildren<QTextEdit*>();
QList<QTextEdit*>::iterator iter = list.begin();
for( ; iter != list.end(); ++iter ) {
(*iter)->clear();
}
}
{
ui->image->clear();
}
}
void WeatherWidget::slotError()
{
displayError("Error", "An error while download occured");
}
void WeatherWidget::slotDone(const QUrl &url, const QByteArray &ba)
{
ui->textEdit->setPlainText(ba);
ui->requestLine->setText(url.toString());
picojson::value v;
std::string error;
picojson::parse(v, ba.constData(), ba.constData()+ ba.size(), &error);
if (!error.empty()) {
displayError("Error", QString("Error occured ") + QString().fromStdString(error));
return;
}
if (!v.is<picojson::object>()) {
displayError("Error", "It is not JSON object");
return;
} else {
JSONParseAndFill(v.get<picojson::object>());
}
}
void WeatherWidget::slotImgDone(const QUrl &, const QByteArray &ba)
{
QPixmap pix;
pix.loadFromData(ba);
ui->image->setPixmap(pix);
QVariant qvar = ui->image->property("fileName");
QString name = qvar.value<QString>();
cache_[name] = ba;
}
void WeatherWidget::slotResetToDefault(bool)
{
ui->APPID->setText(APPID_);
}
void WeatherWidget::slotError(QString errStatus)
{
qDebug() << "Debug message void WeatherWidget::slotError(QString errStatus)";
displayError("Error", errStatus);
}
void WeatherWidget::JSONParseAndFill(picojson::value::object &obj)
{
picojson::value::object::const_iterator i = obj.begin();
for ( ; i != obj.end(); ++i ) {
picojson::value v = i->second;
if (i->second.is<picojson::object>()) {
JSONParseAndFill(v.get<picojson::object>());
} else if (i->second.is<picojson::array>()) {
JSONParseAndFill(v.get<picojson::array>());
} else {
setText(QString().fromStdString(i->first),
QString().fromStdString(i->second.to_str())
);
}
}
}
void WeatherWidget::JSONParseAndFill(picojson::value::array &obj)
{
picojson::value::array::iterator i = obj.begin();
for ( ; i != obj.end(); ++i ) {
picojson::value v = *i;
if ((*i).is<picojson::object>()) {
JSONParseAndFill(v.get<picojson::object>());
} else if ( (*i).is<picojson::array>() ) {
JSONParseAndFill(v.get<picojson::array>());
}
}
}
void WeatherWidget::displayError(const QString &title, const QString& message)
{
QMessageBox::critical(0, title, message);
}
void WeatherWidget::setText(const QString &widgetName, const QString &value)
{
QWidget* curr = ui->layoutWidget->findChild<QWidget*>(widgetName);
if (curr && curr->metaObject()->className() == QString("QLineEdit") ) {
if (curr->objectName() == "icon") {
ui->image->setProperty("fileName", QVariant(value));
downloadImg(value);
}
qobject_cast<QLineEdit*>(curr)->setText(value);
}
}
void WeatherWidget::downloadImg(const QString &imgName)
{
QString request = urlRoot_ + weatherImgQuery_ + imgName + imgExtention_;
qDebug() << request;
const QByteArray* ba = checkCache(imgName);
if( ba != nullptr ) {
qDebug() << request << "From cache";
slotImgDone(QUrl(request), *ba);
} else {
pdlImg_->download(QUrl(request));
}
}
const QByteArray *WeatherWidget::checkCache(const QString &key)
{
ImgCache::const_iterator i = cache_.find(key);
if ( i != cache_.end() ) {
return &(*i);
}
return nullptr;
}
<file_sep>#ifndef CITY_H
#define CITY_H
class City
{
public:
City();
};
#endif // CITY_H
<file_sep>#include <QApplication>
#include "lo_treewidget.h"
#include "weatherwidget.h"
#include <QHBoxLayout>
#include <QWidget>
#include "logger.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
a.setWindowIcon(QIcon(QPixmap(":/icons/mainIcon.png")));
Logger log(Logger::mode::clear);
QWidget central;
#ifdef QT_DEBUG
LO_TreeWidget tree(":/allcities/city.list1.json");
#elif QT_NO_DEBUG
LO_TreeWidget tree(":/allcities/city.list.json");
#else
#error("Please define QT_DEBUG or QT_DEBUG macro")
#endif
if ( !tree.loadData()) {
return -1;
}
tree.show();
//
WeatherWidget weather;
weather.show();
weather.connectLogger(log);
QObject::connect(&tree, SIGNAL(activated(QString)),
&weather, SLOT(slotGo(QString))
);
//Layouts
QHBoxLayout* phbl = new QHBoxLayout();
phbl->addWidget(&tree);
phbl->addWidget(&weather);
central.setLayout(phbl);
central.show();
return a.exec();
}
<file_sep>#ifndef LOGGER_H
#define LOGGER_H
#include <QFile>
#include <QObject>
class Logger : public QObject
{
Q_OBJECT
private:
QString logFileName_;
QFile log_;
public:
struct mode {
enum ENUM { clear, append };
};
explicit Logger( mode::ENUM, QObject* parrent = 0 );
~Logger();
Logger &operator<<(const QString& logThis);
private:
Logger(const Logger& orig) = delete;
Logger& operator=(const Logger& rhs) = delete;
void markStartMessage();
void markEndMessage();
void addNextLine();
public slots:
void slotLog(const QUrl&,const QByteArray&);
void slotLog(const QUrl&);
};
#endif // LOGGER_H
<file_sep>#include "lo_treewidget.h"
#include "data.h"
#include <QByteArray>
#include <QFile>
#include <memory>
#include <iostream>
#include <QDebug>
#include <QStringList>
#include <cassert>
LO_TreeWidget::LO_TreeWidget(const QString& sourceFileName, QWidget *parent) :
QTreeWidget(parent),
sourceFileName_(sourceFileName)
{
setMinimumWidth(200);
QStringList lst;
lst << "Name" << "Id" << "Country" << "Lon" << "Lat";
setHeaderLabels(lst);
setSortingEnabled(true);
}
bool LO_TreeWidget::loadData()
{
QFile file(sourceFileName_);
if ( !file.open(QIODevice::ReadOnly)) {
return false;
} else {
QHash<QChar, QTreeWidgetItem*> firstSymbolHash;
QByteArray b_array(1024, '\0');
std::shared_ptr<City> sp_city;
while ( file.readLine(b_array.data(), 1024) != -1 ) {
sp_city = City::makeCity(b_array);
if ( sp_city.get() != nullptr){
QChar firstCh = sp_city->name().at(0);
QTreeWidgetItem* p_topLevel = 0;
auto iter = firstSymbolHash.find(firstCh);
if ( iter == firstSymbolHash.cend()) {
p_topLevel = new QTreeWidgetItem(this);
p_topLevel->setText(0, firstCh);
firstSymbolHash[firstCh] = p_topLevel;
} else {
p_topLevel = iter.value();
}
assert(p_topLevel!= 0 &&
"bool LO_TreeWidget::loadData(), QTreeWidgetItem* p_topLevel = 0"
);
QTreeWidgetItem* p_twgItem = new QTreeWidgetItem(p_topLevel);
p_twgItem->setText(0, sp_city->name());
p_twgItem->setText(1, QString::number(sp_city->id()));
p_twgItem->setText(2, sp_city->country());
p_twgItem->setText(3, QString::number(sp_city->coord().lon()));
p_twgItem->setText(4, QString::number(sp_city->coord().lat()));
}
}
sortByColumn(0,Qt::AscendingOrder);
}
connect(this,
SIGNAL(itemActivated(QTreeWidgetItem*,int)),
SLOT(slot_emitActivated(QTreeWidgetItem*,int))
);
return true;
}
void LO_TreeWidget::slot_emitActivated(QTreeWidgetItem* item ,int col) {
emit activated(item->text(0));
}
| a170c43aca46dcfde95e7fd9b91d9a66e6fc3c74 | [
"C++"
] | 12 | C++ | markkun/QtWeatherJSONCheck | ca87830bb10f2eaf538722bbb48bd562c96a32ac | dc9a0c58c542921bb45b9d82162c51ecf4d48486 | |
refs/heads/master | <file_sep><?php include("include/include_index.php"); ?>
<style type="text/css">
<!--
body {
margin-left: 0px;
margin-top: 0px;
margin-right: 0px;
margin-bottom: 0px;
}
-->
</style><form name="form1" method="post" action="">
</form>
<file_sep><?php
function jsDebug($strDebug)
{
echo "<script language='JavaScript' type='text/javascript'>alert('";
echo "MySQL Query : $strDebug";
echo "');</script>";
}
//Aceasta clasa permite citirea inregistrarilor
//dintr-o tabela sau interogare MySQL
//Data: 29.11.2006
//Autor: <NAME>
class CTableReader
{
var $m_tableName = null;
var $m_strSQL = null;
var $m_whereClause = null;
var $m_fields = null;
var $m_keys = null;
var $m_results = null;
var $m_rows = null;
var $m_externalQuery = null;
/***************************************************************
CTableReader constructor
arguments: $tableName tyep: string
***************************************************************/
function CTableReader($tableName) {
$this->m_tableName = $tableName;
$this->m_strSQL = "";
$this->m_whereClause = "";
$this->m_fields = array();
$this->m_keys = array();
$this->m_externalQuery = false;
}
function addField($fieldName) {
$this->m_fields[count($this->m_fields)] = $fieldName;
}
function setFilter($key, $value) {
$this->m_keys[$key] = $value;
}
function exec() {
if(!$this->m_externalQuery) {
$this->m_strSQL = "SELECT ";
foreach($this->m_fields as $key => $field) {
if($key > 0) $this->m_strSQL .= ", ";
$this->m_strSQL .= $field;
}
$this->m_strSQL .= " FROM ".$this->m_tableName;
if(count($this->m_keys) > 0) {
$this->m_whereClause = " WHERE ";
$b_first=TRUE;
foreach($this->m_keys as $key => $value) {
if(!$b_first) $this->m_whereClause .= " AND ";
$this->m_whereClause .= $key. "=". $value;
$b_first=FALSE;
}
$this->m_strSQL .= $this->m_whereClause;
}
}
//print_r($this->m_strSQL);
if(!$this->m_results = mysql_query($this->m_strSQL)) {
jsDebug($this->m_strSQL);
}
else {
$this->m_rows = mysql_fetch_array($this->m_results);
}
if($this->m_rows) return TRUE;
return FALSE;
}
function next() {
if($this->m_results )
$this->m_rows = mysql_fetch_array($this->m_results);
if($this->m_rows) return true;
return false;
}
function getValue($fieldName, $with_echo=true) {
if($this->m_rows) {
if($with_echo)
echo $this->m_rows[$fieldName];
else
return $this->m_rows[$fieldName];
}
return null;
}
function setSQL($sqlQuery) {
$this->m_strSQL = $sqlQuery;
$this->m_externalQuery = true;
}
function seek($iRow) {
if($this->m_results)
mysql_data_seek($this->m_results, $iRow);
}
function debug() {
jsDebug($this->m_strSQL);
}
};
//Aceasta clasa permite adaugarea/modificarea inregistrarilor
//dintr-o tabela sau interogare MySQL
//Data: 01.12.2006
class CTableUpdate
{
var $m_tableName = null;
var $m_strSQL = null;
var $m_whereClause = null;
var $m_fields = null;
var $m_keys = null;
var $m_results = null;
/***************************************************************
CTableReader constructor
arguments: $tableName type: string
***************************************************************/
function CTableUpdate($tableName) {
$this->m_tableName = $tableName;
$this->m_strSQL = "";
$this->m_fields = array();
$this->m_keys = array();
}
function addPostString($fieldName,$value) {
$this->m_fields[$fieldName] = "'${_POST[$value]}'";
}
function addPostNumber($fieldName,$value) {
$this->m_fields[$fieldName] = $_POST[$value];
}
function addStringValue($fieldName,$value) {
$this->m_fields[$fieldName] = "'".$value."'";
}
function addNumericValue($fieldName,$value) {
$this->m_fields[$fieldName] = $value;
}
function setFilter($fieldName, $value) {
$this->m_keys[$fieldName] = $value;
}
function formWhereClause() {
if(count($this->m_keys) > 0) {
$this->m_whereClause = " WHERE ";
$b_first=TRUE;
foreach($this->m_keys as $key => $value) {
if(!$b_first) $this->m_whereClause .= " AND ";
$this->m_whereClause .= $key. "=". $value;
$b_first=FALSE;
}
}
}
function exec() {
CTableUpdate::formWhereClause();
$this->m_strSQL = "SELECT * FROM ".$this->m_tableName. $this->m_whereClause;
if($res = mysql_query($this->m_strSQL)) {
if(mysql_num_rows($res) > 0) { // Inregistrarea exista
$this->m_strSQL = "UPDATE ". $this->m_tableName. " SET ";
$b_first=TRUE;
foreach($this->m_fields as $key => $value) {
if(!$b_first) $this->m_strSQL .= ", ";
$this->m_strSQL .= $key. "=". $value;
$b_first=FALSE;
}
if(isset($this->m_whereClause)) {
$this->m_strSQL .= $this->m_whereClause;
}
}
else { // Inregistrarea nu exista
$this->m_strSQL = "INSERT INTO ". $this->m_tableName. " ( ";
$b_first=TRUE;
foreach($this->m_keys as $key => $value) {
if(!$b_first) $this->m_strSQL .= ", ";
$this->m_strSQL .= $key;
$b_first=FALSE;
}
foreach($this->m_fields as $key => $value) {
if(!$b_first) $this->m_strSQL .= ", ";
$this->m_strSQL .= $key;
$b_first=FALSE;
}
$this->m_strSQL .= " ) VALUES ( ";
$b_first=TRUE;
foreach($this->m_keys as $key => $value) {
if(!$b_first) $this->m_strSQL .= ", ";
$this->m_strSQL .= $value;
$b_first=FALSE;
}
foreach($this->m_fields as $key => $value) {
if(!$b_first) $this->m_strSQL .= ", ";
$this->m_strSQL .= $value;
$b_first=FALSE;
}
$this->m_strSQL .= " ) ";
}
}
//print_r($this->m_strSQL);
if(strlen($this->m_strSQL) > 0) {
if(!$this->m_results = mysql_query($this->m_strSQL)) {
jsDebug($this->m_strSQL);
print_r($this->m_strSQL);
}
else {
return TRUE;
}
}
return FALSE;
}
function adauga() {
CTableUpdate::formWhereClause();
// Adauga o inregistrare
$this->m_strSQL = "INSERT INTO ". $this->m_tableName. " ( ";
$b_first=TRUE;
foreach($this->m_keys as $key => $value) {
if(!$b_first) $this->m_strSQL .= ", ";
$this->m_strSQL .= $key;
$b_first=FALSE;
}
foreach($this->m_fields as $key => $value) {
if(!$b_first) $this->m_strSQL .= ", ";
$this->m_strSQL .= $key;
$b_first=FALSE;
}
$this->m_strSQL .= " ) VALUES ( ";
$b_first=TRUE;
foreach($this->m_keys as $key => $value) {
if(!$b_first) $this->m_strSQL .= ", ";
$this->m_strSQL .= $value;
$b_first=FALSE;
}
foreach($this->m_fields as $key => $value) {
if(!$b_first) $this->m_strSQL .= ", ";
$this->m_strSQL .= $value;
$b_first=FALSE;
}
$this->m_strSQL .= " ) ";
//print_r($this->m_strSQL);
if(strlen($this->m_strSQL) > 0) {
if(!$this->m_results = mysql_query($this->m_strSQL)) {
jsDebug($this->m_strSQL);
}
else {
return TRUE;
}
}
return FALSE;
}
function debug() {
jsDebug($this->m_strSQL);
}
};
///include("check_pass.php");
?>
<file_sep><?php
//Conectare la baza de date
$conn = mysql_connect('localhost','nuntala_nick','nicu0712');
if(!$conn) {
echo "Could not connect to mysql server".mysql_error();
die();
}
if(!mysql_select_db('nuntala_book')) {
echo "Could not select nuntala_book".mysql_error();
die();
}
?>
<file_sep><?php
session_start ();
ob_start();
header("Expires: Mon, 26 Jul 1997 05:00:00 GMT"); // date in the past
header("Last-Modified: " . gmdate("D, d M Y H:i:s") . " GMT"); // always modified
header("Cache-Control: no-store, no-cache, must-revalidate"); // HTTP/1.1
header("Cache-Control: post-check=0, pre-check=0", false);
header("Cache-Control: private");
header("Pragma: no-cache"); // HTTP/1.0
if($_SESSION['admin_csm'] == null || $_SESSION['admin_csm'] != 1967)
header( "Location: start.php");
?>
<file_sep><?php //include("file:///D|/_Works/_tipo_4/tipo4/dbscript/check_pass_tip_cont.php"); ?>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1">
<meta name="GENERATOR" content="Microsoft FrontPage 4.0">
<meta name="ProgId" content="FrontPage.Editor.Document">
<title>Magic Weddings || Cartea de oaspeti</title>
<script language="JavaScript" fptype="dynamicanimation">
<!--
function dynAnimation() {}
function clickSwapImg() {}
function MM_findObj(n, d) { //v4.01
var p,i,x; if(!d) d=document; if((p=n.indexOf("?"))>0&&parent.frames.length) {
d=parent.frames[n.substring(p+1)].document; n=n.substring(0,p);}
if(!(x=d[n])&&d.all) x=d.all[n]; for (i=0;!x&&i<d.forms.length;i++) x=d.forms[i][n];
for(i=0;!x&&d.layers&&i<d.layers.length;i++) x=MM_findObj(n,d.layers[i].document);
if(!x && d.getElementById) x=d.getElementById(n); return x;
}
function MM_preloadImages() { //v3.0
var d=document; if(d.images){ if(!d.MM_p) d.MM_p=new Array();
var i,j=d.MM_p.length,a=MM_preloadImages.arguments; for(i=0; i<a.length; i++)
if (a[i].indexOf("#")!=0){ d.MM_p[j]=new Image; d.MM_p[j++].src=a[i];}}
}
function MM_swapImgRestore() { //v3.0
var i,x,a=document.MM_sr; for(i=0;a&&i<a.length&&(x=a[i])&&x.oSrc;i++) x.src=x.oSrc;
}
function MM_swapImage() { //v3.0
var i,j=0,x,a=MM_swapImage.arguments; document.MM_sr=new Array; for(i=0;i<(a.length-2);i+=3)
if ((x=MM_findObj(a[i]))!=null){document.MM_sr[j++]=x; if(!x.oSrc) x.oSrc=x.src; x.src=a[i+2];}
}
//-->
</script>
<script language="JavaScript1.2" fptype="dynamicanimation" src="file:///D|/_Works/_tipo_4/tipo4/animate.js">
</script>
<base target="middle">
<link href="file:///D|/_Works/_tipo_4/tipo4/cases.css" rel="stylesheet" type="text/css">
<style type="text/css">
<!--
.style9 { font-size: 12px;
font-family: Arial, Helvetica, sans-serif;
font-weight: bold;
color: #FFFFFF;
}
.style14 {color: #000099}
.style21 {font-family: Arial, Helvetica, sans-serif; color: #FFFFFF; font-size: 12px;}
body {
background-image: url(back_page.jpg);
}
.style29 {
color: #FFCCFF;
font-family: Arial, Helvetica, sans-serif;
}
.style30 {
font-size: 12px;
font-style: italic;
}
.style31 {font-size: 12px}
-->
</style>
</head>
<?php
include("dbscript/connect.php");
include("dbscript/db_utils.php");
include("dbscript/data_page.php");
if(isset($_POST['date_comment'])) {
if($_POST['date_comment'] == 'adauga') {
$dbUpdate = new CTableUpdate('guest_book');
$dbUpdate->setFilter('nume', "'{$_POST['nume']}'");
$dbUpdate->addPostString('mail','mail');
$dbUpdate->addPostString('mesaj','mesaj');
$dbUpdate->addStringValue('data', date("Y-m-d"));
$dbUpdate->exec();
}
}
$dbGuest = new CTableReader('guest_book');
$qryLista = " select * from guest_book order by guest_id desc";
$dbGuest->setSQL($qryLista);
$dbGuest->exec();
?>
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td width="33%"></td>
<td width="33%">
<table border="0" width="500" cellspacing="0" cellpadding="0">
<tr>
<td width="100%">
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td> <p align="center"><font size="5" face="Verdana" color="#FF99FF">Magic Weddings - Carte de oaspeti </font>
<p align="center"><font size="5" face="Verdana" color="#FF99FF"><br>
</font></b></td>
</tr>
</table>
</td>
</tr>
<tr>
<td width="100%">
<form method="POST" action="carte_oaspeti.php" name="carte_oaspeti">
<!--webbot bot="SaveResults" startspan
U-File="_private/form_results.txt" S-Format="TEXT/CSV"
S-Label-Fields="TRUE" --><input TYPE="hidden" NAME="VTI-GROUP" VALUE="0">
<!--webbot
bot="SaveResults" endspan i-checksum="43374" -->
<table border="0" width="700" cellspacing="0" cellpadding="0">
<tr>
<td colspan="2">
<div align="center"><a href="javascript:window.close();">
</a>
<table width="100%" border="0" cellspacing="0" cellpadding="0">
<tr>
<td><div align="left">
<input name="button01" type=button id="button01" onClick="javascript:top.window.close();" value="Inchide Pagina">
</a></div></td>
<td> </td>
<td><div align="right">
<input name="button02" type=button id="button02" onClick="new_comment()" value="Adauga un Comentariu">
</a></div></td>
</tr>
</table>
��������������������������������������������
</div></td>
</tr>
<tr>
<td> </td>
</tr>
<tr>
<td colspan="2"><p align="center"> </p>
<table width="100%" border="0" cellpadding="0" cellspacing="2">
<tr>
<td><div align="center">
<table width="100%">
<tr>
<td><div align="center"></div></td>
</tr>
</table>
</div></td>
</tr>
<tr>
<?php
$iLinie=0;
while($dbGuest->getValue('guest_id',FALSE)) {
echo "<tr id='trid".$iLinie."'>";
echo "<td><div align='left'>";
echo "<p><span class='style29'><span class='style31'>";
echo $dbGuest->getValue('guest_id', FALSE);
echo ". ";
echo "  ";
echo ymd_to_dmy($dbGuest->getValue('data', FALSE));
echo "</span> | <strong>";
echo $dbGuest->getValue('nume', FALSE);
echo "</strong> <span class='style30'>";
echo "( ";
echo $dbGuest->getValue('mail', FALSE);
echo " )";
echo "</span></span></p>";
echo "<p><span class='style29'>"<em>";
echo $dbGuest->getValue('mesaj', FALSE);
echo "</em>"<br>";
echo "----------------------------------------------------------------------------------------------------------------------------------------- </span><br>";
echo "<p><br>";
echo "</p>";
echo "</div></td>";
echo "</tr>";
$iLinie++;
$dbGuest->next();
}
?>
<td><div align="left">
<p> </p>
<p><br>
</p>
</div></td>
</tr>
</table>
<table width="100%" border="0" cellspacing="0" cellpadding="0">
<tr>
<td>
<input name="button03" type=button id="button03" onClick="javascript:top.window.close();" value="Inchide Pagina">
</a></td>
<td> </td>
<td><div align="right">
<input name="button04" type=button id="button04" onClick="new_comment()" value="Adauga un Comentariu">
</a></div></td>
</tr>
</table> <p align="center"> </p></td>
</tr>
</table>
<div align="center">
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td width="100%"></td>
</tr>
</table>
<div align="center"><a href="#" onMouseOut="MM_swapImgRestore()" onMouseOver="MM_swapImage('Image8','','file:///D|/_Works/_tipo_4/tipo4/images/ajutor2.jpg',1)"><br>
</a></div>
</div>
<span class="style14"> </span>
</div>
</form>
</td>
</tr>
</table>
</td>
<td width="34%"></td>
</tr>
</table>
</body>
</html>
<script language="JavaScript" fptype="dynamicanimation">
function new_comment()
{
var thisForm = document.forms['carte_oaspeti'];
thisForm.action= 'adauga_comentariu.php';
thisForm.target = "_self";
thisForm.submit();
return false;
}
</script>
<file_sep><?php
//returneaza formatul MySQL YYYY-mm-dd
function dmy_to_ymd($dmyDate)
{
$day=0;
$month=0;
$year=0;
list($day, $month, $year) = sscanf($dmyDate, "%d-%d-%d");
$mysql_date = $year."-".$month."-".$day;
return $mysql_date;
}
//Returneaza formatul uman dd-mm-YYYYY
function ymd_to_dmy($ymdDate)
{
$day=0;
$month=0;
$year=0;
list($year, $month, $day) = sscanf($ymdDate, "%d-%d-%d");
$user_date = sprintf("%02d-%02d-%04d", $day, $month, $year);
return $user_date;
}
?><file_sep><?php //include("file:///D|/_Works/_tipo_4/tipo4/dbscript/check_pass_tip_cont.php"); ?>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1">
<meta name="GENERATOR" content="Microsoft FrontPage 4.0">
<meta name="ProgId" content="FrontPage.Editor.Document">
<title>Magic Weddings || Cartea de oaspeti</title>
<script language="JavaScript" fptype="dynamicanimation">
<!--
function dynAnimation() {}
function clickSwapImg() {}
function MM_findObj(n, d) { //v4.01
var p,i,x; if(!d) d=document; if((p=n.indexOf("?"))>0&&parent.frames.length) {
d=parent.frames[n.substring(p+1)].document; n=n.substring(0,p);}
if(!(x=d[n])&&d.all) x=d.all[n]; for (i=0;!x&&i<d.forms.length;i++) x=d.forms[i][n];
for(i=0;!x&&d.layers&&i<d.layers.length;i++) x=MM_findObj(n,d.layers[i].document);
if(!x && d.getElementById) x=d.getElementById(n); return x;
}
function MM_preloadImages() { //v3.0
var d=document; if(d.images){ if(!d.MM_p) d.MM_p=new Array();
var i,j=d.MM_p.length,a=MM_preloadImages.arguments; for(i=0; i<a.length; i++)
if (a[i].indexOf("#")!=0){ d.MM_p[j]=new Image; d.MM_p[j++].src=a[i];}}
}
function MM_swapImgRestore() { //v3.0
var i,x,a=document.MM_sr; for(i=0;a&&i<a.length&&(x=a[i])&&x.oSrc;i++) x.src=x.oSrc;
}
function MM_swapImage() { //v3.0
var i,j=0,x,a=MM_swapImage.arguments; document.MM_sr=new Array; for(i=0;i<(a.length-2);i+=3)
if ((x=MM_findObj(a[i]))!=null){document.MM_sr[j++]=x; if(!x.oSrc) x.oSrc=x.src; x.src=a[i+2];}
}
//-->
</script>
<script language="JavaScript1.2" fptype="dynamicanimation" src="file:///D|/_Works/_tipo_4/tipo4/animate.js">
</script>
<base target="middle">
<link href="file:///D|/_Works/_tipo_4/tipo4/cases.css" rel="stylesheet" type="text/css">
<style type="text/css">
<!--
.style14 {color: #000099}
body {
background-image: url(back_page.jpg);
}
.style38 {
color: #FFCCFF;
font-family: Arial, Helvetica, sans-serif;
font-size: 12px;
}
.style40 {
color: #FFFFFF;
font-weight: bold;
}
a:link {
color: #FFCCFF;
}
a:visited {
color: #FF66FF;
}
a:hover {
color: #FFFFFF;
}
a:active {
color: #FF66FF;
}
-->
</style>
</head>
<?php
include("dbscript/connect.php");
include("dbscript/db_utils.php");
include("dbscript/data_page.php");
/*
if(isset($_POST['date_comment'])) {
if($_POST['date_comment'] == 'adauga') {
$dbUpdate = new CTableUpdate('guest_book');
$dbUpdate->setFilter('nume', "'{$_POST['nume']}'");
$dbUpdate->addPostString('nume','nume');
$dbUpdate->addPostString('e-mail','mail');
$dbUpdate->addPostString('mesaj','mesaj');
$dbUpdate->addStringValue('data', date("Y-m-d"));
$dbUpdate->exec();
}
}
*/
/*
$dbComentarii = new CTableReader('guest_book');
$qryLista = " SELECT * FROM guest_book";
$dbComentarii->setSQL($qryLista);
$dbComentarii->exec();
$nume = $dbComentarii->getValue("nume",FALSE);
$email = $dbComentarii->getValue("e-mail",FALSE);
$mesaj = $dbComentarii->getValue("mesaj",FALSE);
$data = date("Y-m-d");
*/
//////////////////////////////////////////////////
/*
if(isset($_POST['date_comment'])) {
if($_POST['date_comment'] == 'adauga') {
$dbUpdate = new CTableUpdate('guest_book');
//$dbUpdate->setFilter('guest_id', 'guest_id');
//$dbUpdate->setFilter('nume_cerneala', "'{$_POST['nume_cerneala']}'");
$data = date("Y-m-d");
$nume = $_POST['nume'];
$email = $_POST['mail'];
$mesaj = $_POST['mesaj'];
$dbUpdate->addPostString('nume',$nume);
$dbUpdate->addPostString('e-mail',$email);
$dbUpdate->addPostString('mesaj',$mesaj);
$dbUpdate->addPostString('data',dmy_to_ymd($data));
$dbUpdate->exec();
}
}
*/
?>
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td width="33%"></td>
<td width="33%">
<table border="0" width="500" cellspacing="0" cellpadding="0">
<tr>
<td width="100%">
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td> <p align="center"><font size="5" face="Verdana" color="#FF99FF">Magic Weddings - Adauga un comentariu </font>
<p align="center"><font size="5" face="Verdana" color="#FF99FF"><br>
</font></b></td>
</tr>
</table>
</td>
</tr>
<tr>
<td width="100%">
<form method="POST" action="carte_oaspeti.php" name="form_new_comment">
<!--webbot bot="SaveResults" startspan
U-File="_private/form_results.txt" S-Format="TEXT/CSV"
S-Label-Fields="TRUE" --><input TYPE="hidden" NAME="VTI-GROUP" VALUE="0">
<!--webbot
bot="SaveResults" endspan i-checksum="43374" -->
<table border="0" width="700" cellspacing="0" cellpadding="0">
<tr>
<td colspan="2">
<div align="center"><a href="javascript:window.close();">
</a>
<table width="100%" border="1" cellpadding="1" cellspacing="1" bordercolor="#FF66CC">
<tr>
<td width="19%" class="style38"><div align="right">Numele:</div></td>
<td width="81%"><font size="2" face="Arial">
<input name="nume" type="text" value="" size="25">
</font></td>
</tr>
<tr>
<td class="style38"><div align="right">Adresa de email: </div></td>
<td><font size="2" face="Arial">
<input name="mail" type="text" size="25">
</font></td>
</tr>
<tr>
<td valign="top" class="style38"><div align="right">Comentariu:</div></td>
<td><font size="2" face="Arial">
<textarea name="mesaj" cols="60" rows="3"></textarea>
</font></td>
</tr>
</table>
</div></td>
</tr>
<tr>
<td> </td>
</tr>
<tr>
<td colspan="2"><div align="center">
<input name="button2" type=button onClick="validareDate();" value="Adauga">
</a></div>
<table width="100%" border="0" cellspacing="0" cellpadding="0">
<tr>
<td></a></td>
<td> </td>
<td><div align="right"></a></div></td>
</tr>
</table>
<p align="center" class="style38"><a href="carte_oaspeti.php" target="_self"><span class="style40">retur la cartea de oaspeti </span></a><span class="style40"></span></p></td>
</tr>
</table>
<div align="center">
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td width="100%"></td>
</tr>
</table>
<div align="center"><a href="#" onMouseOut="MM_swapImgRestore()" onMouseOver="MM_swapImage('Image8','','file:///D|/_Works/_tipo_4/tipo4/images/ajutor2.jpg',1)"><br>
</a></div>
</div>
<span class="style14"> </span>
</div>
<input type="hidden" name="date_comment">
</form>
</td>
</tr>
</table>
</td>
<td width="34%"></td>
</tr>
</table>
</body>
</html>
<script language="JavaScript" fptype="dynamicanimation">
function validareDate()
{
var thisForm = document.forms['form_new_comment'];
if(thisForm.nume.value.length == 0) {
alert('Toate campurile trebuie completate');
thisForm.nume.focus();
return;
}
if(thisForm.mail.value.length == 0) {
alert('Toate campurile trebuie completate');
thisForm.mail.focus();
return;
}
if(thisForm.mesaj.value.length == 0) {
alert('Toate campurile trebuie completate');
thisForm.mesaj.focus();
return;
}
thisForm.date_comment.value="adauga";
thisForm.target = "_self";
thisForm.submit();
return false;
}
</script>
<file_sep>[general]
index = "Home|/"
despre-noi = "Despre noi|/despre-noi"
servicii = "Servicii|/servicii"
info = "Informatii utile|/info"
guestbook = "Carte de oaspeti|/guestbook"
contact = "Contact|/contact"
[contact]
sidebarAddress[] = "Deva - HD"
sidebarAddress[] = "Str. Mihai Eminescu"
sidebarAddress[] = "Bl. B, Sc. E, Ap. 71, Et. 2"
sidebarPhones[] = "0745.167.940"
sidebarPhones[] = "0729.736.882"
[servicii]
aranjamente-florale = "Aranjamente florale|/aranjamente-florale"
aranjamente-din-baloane = "Aranjamente din Baloane|#"
decoratiuni-masa-festiva = "Decoratiuni Masa Festiva|#"
decoratiuni-salon = "Decoratiuni de Salon|#"
diverse = "Diverse|#"
[servicii_aranjamente-florale]
baloane-cu-heliu = "Baloane cu heliu|/baloane-cu-heliu"
ghirlande = "Ghirlande|/ghirlande"
inimi-din-baloane = "Inimi din baloane|/"
initiale-si-cifre = "Initiale si cifre|/initiale-si-cifre"
jumbo = "Jumbo|/jumbo"
[despre_noi]
servicii[] = decoratiuni sala
servicii[] = huse scaune
servicii[] = decoratiuni biserica
servicii[] = decoratiuni masina
servicii[] = decoratiuni baloane
servicii[] = invitatii
servicii[] = aranjamente mese festive
servicii[] = carduri
servicii[] = aranjamente prezidiu
servicii[] = meniuri
servicii[] = sonorizare
servicii[] = foto, video
<file_sep><?php
session_start ();
ob_start();
header("Expires: Mon, 26 Jul 1997 05:00:00 GMT"); // date in the past
header("Last-Modified: " . gmdate("D, d M Y H:i:s") . " GMT"); // always modified
header("Cache-Control: no-store, no-cache, must-revalidate"); // HTTP/1.1
header("Cache-Control: post-check=0, pre-check=0", false);
header("Cache-Control: private");
header("Pragma: no-cache"); // HTTP/1.0
// citire date din tabela
include("dbscript/connect.php");
include("dbscript/db_utils.php");
/*
$dbLogin = new CTableReader("admin_tab");
$strSQL = sprintf("SELECT * FROM admin_tab WHERE numele='%s' AND parola_user='%s'",
mysql_real_escape_string($_POST['nume']),
sha1(mysql_real_escape_string($_POST['parola'])));
$dbLogin->setSQL($strSQL);
$dbLogin->exec();
jsDebug("2");
jsDebug($dbLogin->getValue('numele',FALSE));
jsDebug($dbLogin->getValue('parola_user',FALSE));
*/
//if($dbLogin->getValue('admin_id',FALSE)) {
if($_POST['nume'] == "cosmina" & $_POST['parola'] == "deva2009") {
$_SESSION['admin_csm'] = 1967;
header( "Location: lista_comentarii.php");
exit();
}
?>
<html>
<script language="JavaScript" fptype="dynamicanimation">
<!--
function dynAnimation() {}
function clickSwapImg() {}
//-->
</script>
<script language="JavaScript1.2" fptype="dynamicanimation" src="../animate.js">
</script>
<link href="../cases.css" rel="stylesheet" type="text/css">
<style type="text/css">
<!--
.style11 { font-family: Arial, Helvetica, sans-serif;
font-size: 12px;
}
.style7 {
font-size: 14px;
color: #000000;
font-weight: bold;
}
.style18 {
font-family: Arial, Helvetica, sans-serif;
font-size: 14px;
font-weight: bold;
color: #FFFFFF;
}
.style20 {
font-family: Arial, Helvetica, sans-serif;
font-size: 16px;
font-weight: bold;
color: #FF0000;
}
body {
background-image: url();
background-color: #D894FC;
}
-->
</style>
<title>Autentificare esuata</title><body topmargin="0" leftmargin="0" onLoad="dynAnimation()">
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td width="33%"></td>
<td width="33%">
<table border="0" width="720" cellspacing="0" cellpadding="0">
<tr>
<td width="150%">
<table border="0" width="100%" cellspacing="0" bordercolorlight="#FFFFFF" bordercolordark="#0099CC" cellpadding="0">
<tr>
<td> </td>
</tr>
<tr>
<td width="100%">
<form method="POST" action="err_no_user.php" name="form_error" >
<!--webbot bot="SaveResults" startspan
U-File="../_private/form_results.txt" S-Format="TEXT/CSV"
S-Label-Fields="TRUE" -->
<!--webbot
bot="SaveResults" endspan i-checksum="43374" -->
<p align="center"><span class="style20">ATENTIE !!! </span><span class="style18"><br>
tentativa neautorizata de intrare in server </span><span class="style11"><br>
<span class="style7">de pe IP-ul: <?php echo $_SERVER['REMOTE_ADDR']; ?> </span></span></p>
</form>
</td>
</tr>
</table>
</td>
</tr>
</table>
</td>
<td width="34%"></td>
</tr>
</table>
</body>
</html><file_sep><?php session_start(); ?>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1">
<meta name="GENERATOR" content="Microsoft FrontPage 4.0">
<meta name="ProgId" content="FrontPage.Editor.Document">
<title>Magic Weddings</title>
<script language="JavaScript" fptype="dynamicanimation">
<!--
function dynAnimation() {}
function clickSwapImg() {}
function MM_preloadImages() { //v3.0
var d=document; if(d.images){ if(!d.MM_p) d.MM_p=new Array();
var i,j=d.MM_p.length,a=MM_preloadImages.arguments; for(i=0; i<a.length; i++)
if (a[i].indexOf("#")!=0){ d.MM_p[j]=new Image; d.MM_p[j++].src=a[i];}}
}
function MM_swapImgRestore() { //v3.0
var i,x,a=document.MM_sr; for(i=0;a&&i<a.length&&(x=a[i])&&x.oSrc;i++) x.src=x.oSrc;
}
function MM_findObj(n, d) { //v4.01
var p,i,x; if(!d) d=document; if((p=n.indexOf("?"))>0&&parent.frames.length) {
d=parent.frames[n.substring(p+1)].document; n=n.substring(0,p);}
if(!(x=d[n])&&d.all) x=d.all[n]; for (i=0;!x&&i<d.forms.length;i++) x=d.forms[i][n];
for(i=0;!x&&d.layers&&i<d.layers.length;i++) x=MM_findObj(n,d.layers[i].document);
if(!x && d.getElementById) x=d.getElementById(n); return x;
}
function MM_swapImage() { //v3.0
var i,j=0,x,a=MM_swapImage.arguments; document.MM_sr=new Array; for(i=0;i<(a.length-2);i+=3)
if ((x=MM_findObj(a[i]))!=null){document.MM_sr[j++]=x; if(!x.oSrc) x.oSrc=x.src; x.src=a[i+2];}
}
//-->
</script>
<script language="JavaScript1.2" fptype="dynamicanimation" src="animate.js">
</script>
<link href="cases.css" rel="stylesheet" type="text/css">
<style type="text/css">
<!--
body {
margin-top: 9px;
background-image: url(back_page.jpg);
}
a:link {
color: #003366;
}
a:visited {
color: #006699;
}
a:hover {
color: #000000;
}
a:active {
color: #000000;
}
.style29 {color: #FFCCFF}
-->
</style>
</head>
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td width="33%"></td>
<td width="33%">
<table border="0" width="720" cellspacing="0" cellpadding="0">
<tr>
<td width="100%"> </td>
</tr>
<tr>
<td width="100%">
<form method="POST" action="err_no_user.php" name="form_logare">
<!--webbot bot="SaveResults" startspan
U-File="../_private/form_results.txt" S-Format="TEXT/CSV"
S-Label-Fields="TRUE" --><input TYPE="hidden" NAME="VTI-GROUP" VALUE="0"><!--webbot
bot="SaveResults" endspan i-checksum="43374" -->
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td width="100%">
<table border="1" width="100%" bordercolorlight="#FFFFFF" cellspacing="1" bordercolordark="#0099CC">
<tr>
<td width="100%">
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td width="33%"></td>
<td width="33%">
<table border="1" width="400" bordercolorlight="#FFFFFF" cellspacing="4" bordercolordark="#0099CC">
<tr>
<td width="43%"><span class="style29"><font face="Arial" size="2">User : </font></span></td>
<td width="57%">
<p align="center"><font size="2" face="Arial"><input name="nume" type="text" class="white_left" size="20" onKeyDown="return onPressEnter(event)">
</font></td>
</tr>
<tr>
<td width="43%"><span class="style29"><font face="Arial" size="2">Parola:</font></span></td>
<td width="57%">
<p align="center"><font size="2" face="Arial"><input name="parola" type="password" class="white_left" size="20"onKeyDown="return onPressEnter(event)">
</font></td>
</tr>
<tr>
<td colspan="2">
</td>
</tr>
<tr>
<td height="41" colspan="2" bgcolor="#9966FF">
<div align="center">
<input name="button23" type=button onClick="onLogare()" value="Logare">
</a>
</div>
</div></td>
</tr>
</table> </td>
<td width="34%"></td>
</tr>
</table> </td>
</tr>
</table>
</td>
</tr>
</table>
<input type='hidden' name='date_generale_utilizator'>
</form>
</td>
</tr>
</table>
</td>
<td width="34%"></td>
</tr>
</table>
</body>
</html>
<script type="text/javascript">
function onLogare()
{
var thisForm = document.forms['form_logare'];
if(thisForm.nume.value.length == 0) {
alert('Introduceti numele (user)');
thisForm.nume.focus();
return;
}
if(thisForm.parola.value.length == 0) {
alert('Introduceti parola');
thisForm.parola.focus();
return;
}
thisForm.date_generale_utilizator.value="logare";
thisForm.submit();
}
function onPressEnter(event)
{
var thisForm = document.forms['form_logare'];
var btn = thisForm.Image5;
if (document.all){
if (event.keyCode == 13) {
event.returnValue=false;
event.cancel = true;
btn.click();
return false;
}
}
else if (document.getElementById){
if (event.which == 13){
event.returnValue=false;
event.cancel = true;
btn.click();
return false;
}
}
else if(document.layers){
if(event.which == 13) {
event.returnValue=false;
event.cancel = true;
btn.click();
return false;
}
}
return true;
}
</script>
<file_sep><?php
session_start ();
ob_start();
header("Expires: Mon, 26 Jul 1997 05:00:00 GMT"); // date in the past
header("Last-Modified: " . gmdate("D, d M Y H:i:s") . " GMT"); // always modified
header("Cache-Control: no-store, no-cache, must-revalidate"); // HTTP/1.1
header("Cache-Control: post-check=0, pre-check=0", false);
header("Cache-Control: private");
header("Pragma: no-cache"); // HTTP/1.0
if($_SESSION['user_id'] == null)
header( "Location: ../log_membri.php");
/*
$strSQL = "select tip_cont from user_account";
$strSQL .= " where user_id = ".$_SESSION['user_id'];
$res = mysql_query($strSQL);
if($res == 0)
header( "Location: ../user/user_account_1.php");
*/
?>
<file_sep><?php
/**
* index.php
*
* @version $1$
* @copyright Temuraru.ro 2012
* @author <NAME>
*/
$dir = dirname(__FILE__);
$GLOBALS['ROOT_DIR'] = realpath($dir . '/..'); // "/home/temuraru/public_html"
define(APPLICATION_PATH, realpath($dir).'/');
set_include_path( $GLOBALS['ROOT_DIR'] . '/library' . PATH_SEPARATOR . APPLICATION_PATH . '/../library' . PATH_SEPARATOR . get_include_path() );
// var_dump(APPLICATION_PATH, realpath($dir));die;
date_default_timezone_set('Europe/Bucharest');
error_reporting(E_ALL ^ E_NOTICE);
ini_set("display_errors", "On");
error_reporting(E_ALL);ini_set("display_errors", "On");
if(!empty($_GET['debug']) || $_SERVER['REMOTE_ADDR'] == '192.168.127.12'){
ini_set('display_errors','On');error_reporting( E_ALL );
}
//var_dump($GLOBALS);die;
require(APPLICATION_PATH . 'library/Smarty/Smarty.class.php');
$smarty = new Smarty();
$smarty->template_dir = APPLICATION_PATH . 'templates/';
$smarty->compile_dir = APPLICATION_PATH . 'templates_c/';
include_once(APPLICATION_PATH . 'include/functions.php');
include_once(APPLICATION_PATH . 'include/Array.php');
$ini_array = parse_ini_file(APPLICATION_PATH . "config/config.ini", true);
$site = $ini_array;
//print_r($ini_array);die;
//$configObject = simplexml_load_file(APPLICATION_PATH . 'public/xml/site.xml');
//$site = array();
//convertXmlObjToArr($configObject, $site);
//var_dump($site);
$allowedPages = array('index', 'despre-noi', 'servicii', 'info', 'guestbook', 'contact', 'index2');
$template = $allowedPages[0];
$request = substr($_SERVER['REQUEST_URI'], 1);
if (in_array($request, $allowedPages)){
$template = $request;
// var_dump($request, in_array($request, $allowedPages)); die;
switch ($template){
case 'galerie':
break;
case 'contact':
//var_dump($site['contact']);die;
break;
default:
break;
}
//var_dump($configObject);die;
}
//var_dump($site, $template);die;
$smarty->assign('template', $template);
$smarty->assign('site', $site);
$smarty->assign('siteMenu', processMenu($site));
$smarty->assign('template', $template);
$smarty->display('index.html');
function processMenu($site = array()) {
$menu = array();
foreach ($site['general'] as $template => $item) {
$parts = explode('|', $item);
$item = array(
'title' => $parts[0],
'href' => $parts[1]
);
$menu[$template] = $item;
}
return $menu;
}
<file_sep><?php include("dbscript/check_pass_admin.php"); ?>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1">
<meta name="GENERATOR" content="Microsoft FrontPage 4.0">
<meta name="ProgId" content="FrontPage.Editor.Document">
<title>Magic Weddings || Cartea de oaspeti - Lista de Comentarii</title>
<script language="JavaScript" fptype="dynamicanimation">
<!--
function dynAnimation() {}
function clickSwapImg() {}
function MM_findObj(n, d) { //v4.01
var p,i,x; if(!d) d=document; if((p=n.indexOf("?"))>0&&parent.frames.length) {
d=parent.frames[n.substring(p+1)].document; n=n.substring(0,p);}
if(!(x=d[n])&&d.all) x=d.all[n]; for (i=0;!x&&i<d.forms.length;i++) x=d.forms[i][n];
for(i=0;!x&&d.layers&&i<d.layers.length;i++) x=MM_findObj(n,d.layers[i].document);
if(!x && d.getElementById) x=d.getElementById(n); return x;
}
function MM_preloadImages() { //v3.0
var d=document; if(d.images){ if(!d.MM_p) d.MM_p=new Array();
var i,j=d.MM_p.length,a=MM_preloadImages.arguments; for(i=0; i<a.length; i++)
if (a[i].indexOf("#")!=0){ d.MM_p[j]=new Image; d.MM_p[j++].src=a[i];}}
}
function MM_swapImgRestore() { //v3.0
var i,x,a=document.MM_sr; for(i=0;a&&i<a.length&&(x=a[i])&&x.oSrc;i++) x.src=x.oSrc;
}
function MM_swapImage() { //v3.0
var i,j=0,x,a=MM_swapImage.arguments; document.MM_sr=new Array; for(i=0;i<(a.length-2);i+=3)
if ((x=MM_findObj(a[i]))!=null){document.MM_sr[j++]=x; if(!x.oSrc) x.oSrc=x.src; x.src=a[i+2];}
}
//-->
</script>
<script language="JavaScript1.2" fptype="dynamicanimation" src="file:///D|/_Works/_tipo_4/tipo4/animate.js">
</script>
<base target="middle">
<link href="file:///D|/_Works/_tipo_4/tipo4/cases.css" rel="stylesheet" type="text/css">
<style type="text/css">
<!--
.style14 {color: #000099}
body {
background-image: url(back_page.jpg);
}
.style21 {font-family: Arial, Helvetica, sans-serif; color: #FFFFFF; font-size: 12px;}
.style36 {color: #FFFFFF}
.style38 {
color: #FFCCFF;
font-family: Arial, Helvetica, sans-serif;
font-size: 12px;
}
.style40 {color: #FFCCFF}
a:link {
color: #FFCCFF;
}
a:visited {
color: #FF66FF;
}
a:hover {
color: #FFFFFF;
}
a:active {
color: #FF66FF;
}
-->
</style>
</head>
<?php
include("dbscript/connect.php");
include("dbscript/db_utils.php");
include("dbscript/data_page.php");
//STERGERE
if(isset($_POST['rowDel'])) {
$strSQL = "DELETE FROM guest_book WHERE guest_id=".$_POST['rowDel'];
mysql_query($strSQL);
}
$dbGuest = new CTableReader('guest_book');
$qryLista = " select * from guest_book order by guest_id desc";
$dbGuest->setSQL($qryLista);
$dbGuest->exec();
?>
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td width="33%"></td>
<td width="33%">
<table border="0" width="500" cellspacing="0" cellpadding="0">
<tr>
<td width="100%">
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td> <p align="center"><font size="5" face="Verdana" color="#FF99FF">Magic Weddings - Lista Comentarii </font>
<p align="center"><font size="5" face="Verdana" color="#FF99FF"><br>
</font></b></td>
</tr>
</table>
</td>
</tr>
<tr>
<td width="100%">
<form method="POST" action="lista_comentarii.php" name="lista_carte_oaspeti">
<!--webbot bot="SaveResults" startspan
U-File="_private/form_results.txt" S-Format="TEXT/CSV"
S-Label-Fields="TRUE" --><input TYPE="hidden" NAME="VTI-GROUP" VALUE="0">
<!--webbot
bot="SaveResults" endspan i-checksum="43374" -->
<table border="0" width="700" cellspacing="0" cellpadding="0">
<tr>
<td colspan="2">
<div align="center"><a href="javascript:window.close();">
</a>
<table width="100%" border="0" cellspacing="0" cellpadding="0">
<tr>
<td><div align="center"><a href="javascript:window.close();"></a>
<table width="848" border="1" cellpadding="1" cellspacing="1" bordercolorlight="#FFFFFF" bordercolordark="#0099CC" bgcolor="#FFCCFF">
<tr>
<td width="39" height="32" align="center" bgcolor="#660066" class="style21"><span class="style7"><span class="style21">ID</span></span></td>
<td width="80" align="center" bgcolor="#660066" class="style21"><span class="style36">Data</span></td>
<td width="178" align="center" bgcolor="#660066" class="style21">User</td>
<td width="123" align="center" bgcolor="#660066" class="style21">e-mail</td>
<td width="342" align="center" bgcolor="#660066" class="style21"><span class="style7"><span class="style21">Comentariu</span></span></td>
<td width="53" align="center" bgcolor="#660066" class="style21"><div align="center" class="style21">Sterge</div></td>
</tr>
<?php
$iLinie=0;
while($dbGuest->getValue('guest_id',FALSE)) {
echo "<tr id='trid".$iLinie."'>";
echo " <td width='' bgcolor=";
if($iLinie %2) echo "'FFCCFF'>";
else echo "'FFF0FF'>";
echo "<p align='center'><font size='2' face='Arial' color='#560C72'>";
echo $dbGuest->getValue('guest_id', FALSE);
echo "</font>";
echo "</td>";
echo " <td width='' bgcolor=";
if($iLinie %2) echo "'FFCCFF'>";
else echo "'FFF0FF'>";
echo "<p align='center'><font size='2' face='Arial' color='#560C72'>";
echo ymd_to_dmy($dbGuest->getValue('data', FALSE));
echo "</font>";
echo "</td>";
echo " <td width='' bgcolor=";
if($iLinie %2) echo "'FFCCFF'>";
else echo "'FFF0FF'>";
echo "<p align='center'><font size='2' face='Arial' color='#560C72'>";
echo $dbGuest->getValue('nume', FALSE);
echo "</font>";
echo "</td>";
echo " <td width='' bgcolor=";
if($iLinie %2) echo "'FFCCFF'>";
else echo "'FFF0FF'>";
echo "<p align='center'><font size='2' face='Arial' color='#560C72'>";
echo $dbGuest->getValue('mail', FALSE);
echo "</font>";
echo "</td>";
echo " <td width='' bgcolor=";
if($iLinie %2) echo "'FFCCFF'>";
else echo "'FFF0FF'>";
echo "<p align='center'><font size='2' face='Arial' color='#560C72'>";
echo $dbGuest->getValue('mesaj', FALSE);
echo "</font>";
echo "</td>";
echo " <td width='' bgcolor=";
if($iLinie %2) echo "'FFCCFF'>";
else echo "'FFF0FF'>";
$rowId = $dbGuest->getValue('guest_id', FALSE);
echo "<p align='center'><img src='delete_1.png' onClick='return onDelete($rowId)' border='0'>";
echo "</td>";
echo "</font></font></tr>";
$iLinie++;
$dbGuest->next();
}
?>
</table>
</div>
<div align="right"><a href="javascript:window.close();"></a></div></td>
</tr>
</table>
</div></td>
</tr>
<tr>
<td> </td>
</tr>
<tr>
<td colspan="2"><p align="center"> <span class="style14"><a href="#" target="_self" onMouseOver="MM_swapImage('Image7','','file:///D|/_Works/_tipo_4/tipo4/oferta/img/lista_calculatii_2.jpg',1)" onMouseOut="MM_swapImgRestore()"></a>
</span></p>
<table width="100%" border="0" cellspacing="0" cellpadding="0">
<tr>
<td>
<input name="button23" type=button onClick="javascript:top.window.close();" value="Inchide Pagina">
</a></td>
<td> </td>
<td><div align="right"><a href="javascript:window.close();"></a></div></td>
</tr>
</table>
<p align="center" class="style38"><span class="style40"><a href="../index.html" target="_self">homepage</a> | <a href="carte_oaspeti.php" target="_self">guestbook </a></span></p></td>
</tr>
</table>
<div align="center">
<table border="0" width="100%" cellspacing="0" cellpadding="0">
<tr>
<td width="100%"></td>
</tr>
</table>
<div align="center"><a href="#" onMouseOut="MM_swapImgRestore()" onMouseOver="MM_swapImage('Image8','','file:///D|/_Works/_tipo_4/tipo4/images/ajutor2.jpg',1)"><br>
</a></div>
</div>
<span class="style14"> </span>
</div>
<input type="hidden" name="rowDel">
</form>
</td>
</tr>
</table>
</td>
<td width="34%"></td>
</tr>
</table>
</body>
</html>
<script language="JavaScript" fptype="dynamicanimation">
function onDelete(rowId)
{
var thisForm = document.forms['lista_carte_oaspeti'];
if(confirm('Sunteti sigur ca doriti sa stergeti?')) {
thisForm.rowDel.value=rowId;
thisForm.target = "_self";
thisForm.submit();
}
}
</script>
| 3db82935111501870a6f7996be24828655b06281 | [
"PHP",
"INI"
] | 13 | PHP | temuraru/nuntalacheie | 812730fd0d114ce15de50995b20f35a8e69a9bca | 6eb8504b2e5551d6bc755019fc7165e61707c271 | |
refs/heads/master | <repo_name>KienbMi/csharp_livecoding_ef_uow_webapi-part1<file_sep>/source/MovieManager.Web/Controllers/CategoriesController.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using MovieManager.Core.Contracts;
using MovieManager.Core.DataTransferObjects;
using MovieManager.Core.Entities;
namespace MovieManager.Web.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class CategoriesController : ControllerBase
{
private readonly IUnitOfWork _unitOfWork;
public CategoriesController(IUnitOfWork unitOfWork)
{
_unitOfWork = unitOfWork;
}
/// <summary>
/// Liefert alle Kategorien.
/// </summary>
[HttpGet]
[ProducesResponseType(StatusCodes.Status200OK)]
public ActionResult<string[]> GetCategories()
{
return Ok(_unitOfWork
.CategoryRepository
.GetAll()
.Select(category => category.CategoryName)
.ToArray());
}
/// <summary>
/// Liefert eine Kategorie per Id.
/// </summary>
[HttpGet]
[Route("{id}")]
[ProducesResponseType(StatusCodes.Status200OK)]
[ProducesResponseType(StatusCodes.Status400BadRequest)]
[ProducesResponseType(StatusCodes.Status404NotFound)]
public ActionResult<CategoryWithMoviesDto> GetById(int id)
{
Category category = _unitOfWork.CategoryRepository.GetByIdWithMovies(id);
if (category == null)
{
return NotFound();
}
return Ok(new CategoryWithMoviesDto(category));
}
/// <summary>
/// Liefert die Movies zu einer Kategorie (per Id).
/// </summary>
[HttpGet]
[Route("{id}/movies")]
[ProducesResponseType(StatusCodes.Status200OK)]
[ProducesResponseType(StatusCodes.Status400BadRequest)]
[ProducesResponseType(StatusCodes.Status404NotFound)]
public ActionResult<MovieDto[]> GetMoviesByCategoryId(int id)
{
Category category = _unitOfWork.CategoryRepository.GetByIdWithMovies(id);
if (category == null)
{
return NotFound();
}
return Ok(new CategoryWithMoviesDto(category).Movies);
}
}
}
| 4210f9ea68067a0e3cae7569a25b684b09968ec5 | [
"C#"
] | 1 | C# | KienbMi/csharp_livecoding_ef_uow_webapi-part1 | aef26a8a28e9412377e1fcc3ec647a48ca64c78e | f51dbcb9d4c85cb4ce4e773d7e0427d35f87853a | |
refs/heads/master | <file_sep>import gulp from 'gulp';
import gulpLoadPlugins from 'gulp-load-plugins';
import browserSync from 'browser-sync';
import del from 'del';
import browserify from 'browserify';
import debowerify from 'debowerify';
import source from 'vinyl-source-stream';
import mergeStream from 'merge-stream';
import { stream as wiredep } from 'wiredep';
const $ = gulpLoadPlugins();
const reload = browserSync.reload;
var Config = {
path: {
'assets': 'app/assets',
'build': 'web/assets'
},
scripts: ['app.js'],
reload: {
'proxy': 'local.dev/app_dev.php',
'port': 3000
}
};
// Styles
gulp.task('styles', ['wiredep'], () => {
return gulp.src(Config.path.assets + '/styles/*.scss')
.pipe($.plumber({
errorHandler: $.notify.onError('Error: <%= error.message %>')
}))
.pipe($.sourcemaps.init())
.pipe($.sass.sync({
outputStyle: 'expanded',
precision: 10,
includePaths: ['.']
}))
.pipe($.autoprefixer({
browsers: ['> 1%', 'last 2 versions', 'Firefox ESR']
}))
.pipe($.sourcemaps.write())
.pipe(gulp.dest(Config.path.build + '/css'))
.pipe(reload({
stream: true
}));
});
// Scripts
gulp.task('scripts', () => {
var merged = mergeStream();
var scripts = Config.scripts;
scripts.forEach(function(file) {
merged.add(browserify(Config.path.assets + '/scripts/' + file)
.transform(debowerify)
.bundle()
.on('error', $.notify.onError(function(error) {
return error.message;
}))
.pipe(source(file))
.pipe(gulp.dest(Config.path.build + '/js')));
});
return merged;
});
// Lint
function lint(files, options) {
return () => {
return gulp.src(files)
.pipe(reload({
stream: true,
once: true
}))
.pipe($.eslint(options))
.pipe($.eslint.format())
.pipe($.if(!browserSync.active, $.eslint.failAfterError()))
.on('error', $.notify.onError(function(error) {
return 'Linting: ' + error.message;
}));
};
}
gulp.task('lint', lint(Config.path.assets + '/scripts/**/*.js'));
// Assets
gulp.task('assets', ['styles', 'scripts'], () => {
return gulp.src([
Config.path.build + '/js/**/*.js',
Config.path.build + '/css/**/*.css'
], {
base: Config.path.build
})
.pipe($.if('*.js', $.uglify()))
.pipe($.if('*.css', $.cssnano()))
.pipe(gulp.dest(Config.path.build));
});
// Images
gulp.task('images', () => {
return gulp.src(Config.path.assets + '/images/**/*')
.pipe($.cache($.imagemin({
progressive: true,
interlaced: true,
// don't remove IDs from SVGs, they are often used
// as hooks for embedding and styling
svgoPlugins: [{
cleanupIDs: false
}]
})))
.pipe(gulp.dest(Config.path.build + '/img'));
});
// Fonts
gulp.task('fonts', () => {
return gulp.src(require('main-bower-files')('**/*.{eot,svg,ttf,woff,woff2}')
.concat(Config.path.assets + '/fonts/**/*'))
.pipe(gulp.dest(Config.path.build + '/fonts'));
});
// Clean
gulp.task('clean', del.bind(null, [Config.path.build]));
// Serve
gulp.task('serve', ['styles', 'scripts', 'fonts'], () => {
browserSync({
notify: false,
port: Config.reload.port,
proxy: Config.reload.proxy
});
gulp.watch([
'app/Resources/**/*.html.twig',
// 'src/**/Resources/views/**/*.html.twig',
Config.path.build + '/js/**/*.js',
Config.path.build + '/css/**/*.css',
Config.path.build + '/img/**/*',
Config.path.build + '/fonts/**/*'
]).on('change', reload);
gulp.watch(Config.path.assets + '/styles/**/*.scss', ['styles']);
gulp.watch(Config.path.assets + '/scripts/**/*.js', ['scripts']);
gulp.watch(Config.path.assets + '/fonts/**/*', ['fonts']);
gulp.watch(Config.path.assets + '/images/**/*', ['images']);
gulp.watch('bower.json', ['wiredep', 'fonts']);
});
// Wiredep
gulp.task('wiredep', () => {
gulp.src(Config.path.assets + '/styles/*.scss')
.pipe(wiredep())
.pipe(gulp.dest(Config.path.assets + '/styles'));
});
// Build
gulp.task('build', ['lint', 'assets', 'images', 'fonts'], () => {
return gulp.src(Config.path.build + '/**/*').pipe($.size({
title: 'build',
gzip: true
}));
});
// Default task
gulp.task('default', ['clean'], () => {
gulp.start('build');
});
<file_sep>console.log('app.js');
var jQuery = require('jquery');
(function($) {
$('body').append('jQuery should works fine!');
})(jQuery);
<file_sep># gulp-symfony
Symfony assets management with Gulp - made simple.
## About
Speed up your frontend workflow while developing Symfony apps with tools like [Gulp](http://gulpjs.com), [Bower](http://bower.io), [Browserify](http://browserify.org), [Sass](http://sass-lang.com), [BrowserSync](https://browsersync.io) and more. Inspired by and partially copied from [Yeoman](http://yeoman.io) [Web app generator](https://github.com/yeoman/generator-gulp-webapp).
## Features
- Livereload with [BrowserSync](https://browsersync.io)
- CSS with Sass, Autoprefixer, sourcemaps for developing and CSSNano for minification
- JavaScript with [ESLint](http://eslint.org) and [Browserify](http://browserify.org) + [Debowerify](https://github.com/eugeneware/debowerify) for bundling bower components.
- Image optimalization with [imagemin](https://github.com/imagemin/imagemin)
## Installation
Download this repository to your Symfony project folder. Assets are located under app/assets folder, feel free to change if needed.
Install Gulp and Bower globally
```
$ npm install -g gulp bower
```
Install dependencies
```
$ npm install
```
## Configuration
Inside gulpfile you can find Config variable.
```javascript
var Config = {
path: {
'assets': 'app/assets',
'build': 'web/assets'
},
scripts: ['app.js'],
reload: {
'proxy': 'local.dev/app_dev.php',
'port': 3000
}
};
```
`path`: files location, `assets` for source files and `build` for build
`scripts`: you can define more than just one script if you need admin.js or whatever
`reload`: browsersync options, set proxy to your local url
**Important!** Build folder is cleaned before every build, so do not change this folder to web or any other. You don't want to lose it. :)
## Usage
Install vendors with Bower (default Bootstrap 4)
```
$ bower install
```
`wiredep` task automatically inject files, using main entry inside bower.json, for overriding main entry see [wiredep docs](https://github.com/taptapship/wiredep/tree/master#bower-overrides)
For imported scss files use name prefixed with underscore, because Sass does not compile those files.
Gulp tasks
```
$ gulp -T
```
Build
```
$ gulp
```
Develop
```
$ gulp serve
```
For more information check docs of the tools used in gulpfile.
## Symfony
Linking in Twig
```html
<link rel="stylesheet" href="{{ asset('assets/css/app.css') }}">
<script src="{{ asset('assets/js/app.js') }}"></script>
```
**Note**
By default BrowserSync reloads only if views in app folder changed, you can enable this also for bundle views. Just simply [uncomment this line](https://github.com/makao/gulp-symfony/blob/master/gulpfile.babel.js#L131) in gulpfile.
```javascript
// 'src/**/Resources/views/**/*.html.twig',
``` | e2c2e7d1897b7c5c4fdc555608716a4af52c70c8 | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | makao/gulp-symfony | 5e1b2f151ed003709c739ba9df5b499cfb7d26c6 | 943a79762df555619222d22024a1c8d175229922 | |
refs/heads/master | <repo_name>JosyAnjos/blog2_app<file_sep>/app/helpers/articles_helper.rb
module ArticlesHelper
def persisted_comments(comments)
comments.reject{|comment|comment.new_record?}
end
end
| 062601ac3d1dbecea5474bdcd808aa5b16fa6f91 | [
"Ruby"
] | 1 | Ruby | JosyAnjos/blog2_app | a427f7b8f199c5606563085bbb366d3ebc3263c4 | bbdbff2a7ca6e2be4fafb617a0ab8f45f71123a7 | |
refs/heads/main | <file_sep># OpenWeatherMap API Key
weather_api_key = "00e181b4e35294370a45258f71514f3c"
# Google API Key
g_key = "<KEY>"
| 201c567a9f0e2c8dc6301bc0a22f737a0dd7ab5f | [
"Python"
] | 1 | Python | emily-wuenstel/python-api-challenge | f494663be554e4cc314349ddfa19d5cd0484778c | e799e3becf3b0735c8611410f33e668427c5e1e5 | |
refs/heads/master | <file_sep>/**
* # Popup
*
* 对话框.
*/
/**
* @require [core](./core.js.html)
* @require [Lite](./lite.js.html)
*/
var core = require('./core'),
$ = require('./lite'),
template = require('./tpl/popup')
/**
* ## Popup Constructor
*
* Popup 构造函数。
*
* 使用:
* ```js
* var popup = new Kub.Popup({
* message:'popup dialog'
* })
* ```
*/
function Popup(options) {
this.options = core.extend({}, _prototype.defaults, options || {})
init(this)
}
var ANIMATION_CLASS = 'kub-popup-animation',
EVENT_NAME = 'click',
POPUP_SELECTOR = '.J_popup'
var $body
var _prototype = Popup.prototype
var render = function(popup,data) {
var html = template(data)
popup.$element = $(html).appendTo($body)
return this
}
var bindEvents = function(popup){
popup.$element.on(EVENT_NAME, function(e) {
if($(e.target).closest(POPUP_SELECTOR).length){
return
}
popup.close()
})
}
var init = function(popup) {
!$body && ($body = $(document.body))
//渲染数据
render(popup, popup.options)
bindEvents(popup)
popup.show()
}
/**
* ## Popup.prototype.defaults
*
* 默认配置项。
*
* 配置项说明:
*
* * `message` : `String` 弹窗内容。
*
* * `className` : `String` 弹窗类名。
*
*/
_prototype.defaults = {
message: '',
className: '',
duration:350
}
/**
* ## Popup.prototype.show
*
* 显示弹窗。
* @return {instance} 返回当前实例
*/
_prototype.show = function() {
clearTimeout(this._timer)
this.$element.show().addClass(ANIMATION_CLASS)
return this
}
/**
* ## Popup.prototype.hide
*
* 隐藏弹窗。
* @return {instance} 返回当前实例
*/
_prototype.hide = function(callback) {
var self = this,
$element = self.$element
function handler(){
$element.hide()
callback && callback()
}
$element.removeClass(ANIMATION_CLASS)
clearTimeout(self._timer)
self._timer = setTimeout(function(){
handler()
},self.options.duration)
return self
}
/**
* ## Popup.prototype.close
*
* 关闭弹窗。
* @return {instance} 返回当前实例
*/
_prototype.close = function() {
var self = this,
opts = self.options
if (opts.closeHandler && opts.closeHandler.call(self) === false) {
return self
}
self.hide(function(){
self.$element.remove()
})
return self
}
module.exports = Popup
<file_sep>/**
* # Lite
*
* 类似于`Zepto`,提供部分与Dom相关的方法,方法使用保持与`Zepto`一致。
*
*/
/**
* @require [polyfill](./polyfill.js.html)
*/
require('./polyfill')
var $, Lite
var ELEMENT_NODE = 1
var slice = Array.prototype.slice,
readyRE = /complete|loaded|interactive/,
idSelectorRE = /^#([\w-]+)$/,
classSelectorRE = /^\.([\w-]+)$/,
tagSelectorRE = /^[\w-]+$/,
spaceRE = /\s+/g
var _document = document,
_window = window,
_undefined = undefined
function wrap(dom, selector) {
dom = dom || []
Object.setPrototypeOf ? Object.setPrototypeOf(dom, $.fn) : (dom.__proto__ = $.fn)
dom.selector = selector || ''
return dom
}
var isArray = Array.isArray ||
function(object) {
return object instanceof Array
}
$ = Lite = function(selector, context) {
context = context || _document
var type = typeof selector
if (!selector) {
return wrap()
}
if (type === 'function') {
return $.ready(selector)
}
if (selector._l) {
return selector
}
if (isArray(selector)) {
//$([document,document.body])
return wrap(slice.call(selector).filter(function(item) {
return item != null
}), selector)
}
if (type === 'object') {
//$(document)
return wrap([selector], '')
}
if (type === 'string') {
selector = selector.trim()
if (selector[0] === '<') {
var nodes = $.fragment(selector)
return wrap(nodes, '')
}
if (idSelectorRE.test(selector)) {
var found = _document.getElementById(RegExp.$1)
return wrap(found ? [found] : [], selector)
}
return wrap($.qsa(selector, context), selector)
}
return wrap()
}
var createDelegator = function(handler, selector, element) {
return function(e) {
var match = $(e.target).closest(selector)
// 1、存在代理节点
// 2、排除$('.className').on('click','.className',handler) 情况
if (match.length && match[0] !== element) {
handler.apply(match[0], arguments)
}
}
}
!(function() {
this.qsa = function(selector, context) {
//querySelectorAll,如果存在两个相同ID,在ios7下,限定范围内查询 id 会返回两个节点
context = context || _document
selector = selector.trim()
return slice.call(classSelectorRE.test(selector) ? context.getElementsByClassName(RegExp.$1) : tagSelectorRE.test(selector) ? context.getElementsByTagName(selector) : context.querySelectorAll(selector))
}
this.fragment = function(html) {
var div = _document.createElement('div'),
nodes
div.innerHTML = html
nodes = div.children
div = null
return slice.call(nodes)
}
this.ready = function(callback) {
if (readyRE.test(_document.readyState) && _document.body) {
callback($)
} else {
_document.addEventListener('DOMContentLoaded', function() {
callback($)
}, false)
}
return this
}
this.Event = function(type, props) {
return new _window.CustomEvent(type, props)
}
this.fn = this.prototype = {
_l: true,
/**
* ## Lite.prototype.each
*
* 循环所有节点。
*/
each: function(callback) {
var l = this.length,
i, t
for (i = 0; i < l; i++) {
t = this[i]
if (callback.call(t, i, t) === false) {
return this
}
}
return this
},
/**
* ## Lite.prototype.slice
*
* 切割节点。
*/
slice: function() {
return $(slice.apply(this, arguments))
},
/**
* ## Lite.prototype.is
*
* 判断是否是指定的节点。
*/
is: function(selector, element) {
element = element ? element : this[0]
if (element && element.nodeType === ELEMENT_NODE) {
return element === selector ? true : typeof selector === 'string' && element.matches(selector)
}
return false
},
/**
* ## Lite.prototype.closest
*
* 查找最近的节点。
*
* 原生closest不包含本身,`jQuery`与`Zepto`包含本身,保持与`Zepto`一致。
*
*/
closest: function(selector) {
var element = this[0],dom
dom = element && typeof selector === 'string' ? element.closest(selector) : this.is(selector, element) ? element : null
return $(dom)
},
/**
* ## Lite.prototype.find
*
* 查找节点,只支持选择器查找.
*/
find: function(selector) {
var dom = []
this.each(function() {
if (this.nodeType !== ELEMENT_NODE) return
var elements = $.qsa(selector, this),
elementsLen = elements.length
for (var i = 0; i < elementsLen; i++) {
dom.indexOf(elements[i]) === -1 && dom.push(elements[i])
}
})
return $(dom)
},
/**
* ## Lite.prototype.show
*
* 显示。
*/
show: function() {
return this.each(function() {
this.style.display === 'none' && (this.style.display = '')
$(this).css('display') === 'none' && (this.style.display = 'block')
})
},
/**
* ## Lite.prototype.hide
*
* 隐藏。
*/
hide: function() {
return this.each(function() {
this.style.display = 'none'
})
},
/**
* ## Lite.prototype.css
*
* 修改或获取节点样式。
*/
css: function(property, value) {
var isObject = typeof property === 'object'
//get
if (value == null && !isObject) {
var el = this[0]
//not element
if (el.nodeType !== ELEMENT_NODE) return
return _window.getComputedStyle(el).getPropertyValue(property)
}
var css = ''
if (isObject) {
for (var key in property) {
property[key] == null ? this.each(function() {
this.style.removeProperty(key)
}) : (css += key + ':' + property[key] + ';')
}
} else {
css += key + ':' + property[key] + ';'
}
//set
css && this.each(function() {
this.style.cssText += ';' + css
})
return this;
},
/**
* ## Lite.prototype.offset
*
* 获取节点的`offset`,只支持获取,不支持设置。
*/
offset: function() {
if (!this.length) return null
var ele = this[0],
obj = ele.getBoundingClientRect()
//why window.pageXOffset
//http://www.cnblogs.com/hutaoer/archive/2013/02/25/3078872.html
return {
left: obj.left + _window.pageXOffset,
top: obj.top + _window.pageYOffset,
width: ele.offsetWidth,
height: ele.offsetHeight
}
},
/**
* ## Lite.prototype.addClass
*
* 添加`class`。
*/
addClass: function(name) {
if (name == _undefined) return this
return this.each(function() {
if (!('className' in this)) return
var classList = [],
className = this.className.trim(),
classNameList = className.split(spaceRE)
name.trim().split(spaceRE).forEach(function(klass) {
classNameList.indexOf(klass) === -1 && classList.push(klass)
})
classList.length && (this.className = (className && (className + ' ')) + classList.join(' '))
})
},
/**
* ## Lite.prototype.removeClass
*
* 移除`class`。
*/
removeClass: function(name) {
return this.each(function() {
if (!('className' in this)) return
if (name === _undefined) return this.className = ''
var className = this.className
name.trim().split(spaceRE).forEach(function(klass) {
//zepto
className = className.replace(new RegExp('(^|\\s)' + klass + '(\\s|$)', 'g'), ' ')
})
this.className = className
})
},
/**
* ## Lite.prototype.eq
*
* 获取指定索引节点。
*/
eq: function(idx) {
idx = idx < 0 ? idx + this.length : idx
return $(this[idx])
},
/**
* ## Lite.prototype.off
*
* 取消绑定事件,不支持移除代理事件。
*/
off: function(type, handler) {
var types = type && type.trim().split(spaceRE)
types && this.each(function() {
var element = this
types.forEach(function(name) {
if (handler) {
element.removeEventListener(name, handler.delegator || handler, false)
} else {
var handlers = element.listeners && element.listeners[name]
handlers && handlers.forEach(function(_handler) {
element.removeEventListener(name, _handler.delegator || _handler, false)
})
}
})
})
return this
},
/**
* ## Lite.prototype.on
*
* 监听事件,支持事件代理。
*
*/
on: function(type, selector, handler) {
var f = true
if (typeof selector !== 'string') {
f = false
handler = selector
}
if (typeof handler == 'function') {
var types = type && type.trim().split(spaceRE)
types && this.each(function() {
var element = this, listeners
if (f) {
handler.delegator = createDelegator(handler, selector, element)
}
listeners = element.listeners || {}
types.forEach(function(event) {
if (!listeners[event]) {
listeners[event] = []
}
listeners[event].push(handler)
element.addEventListener(event, handler.delegator || handler, false)
})
element.listeners = listeners
})
}
return this
},
/**
* ## Lite.prototype.trigger
*
* 触发事件。
*/
trigger: function(type, detail) {
return this.each(function() {
this && this.dispatchEvent && this.dispatchEvent($.Event(type, {
detail: detail,
bubbles: true,
cancelable: true
}))
})
},
/**
* ## Lite.prototype.attr
*
* 获取或者设置节点属性。
*/
attr: function(name, value) {
var result,
type = typeof name
if(type === 'string' && value == null) {
if(!this.length || this[0].nodeType !== ELEMENT_NODE){
return _undefined
}else{
return (!(result = this[0].getAttribute(name)) && name in this[0]) ? this[0][name] : result
}
}else{
return this.each(function() {
if (this.nodeType !== ELEMENT_NODE) return
if (type === 'object') {
for (key in name) {
this.setAttribute(key, name[key])
}
} else {
this.setAttribute(name, value)
}
})
}
},
/**
* ## Lite.prototype.removeAttr
*
* 移除节点属性。
*/
removeAttr: function(name) {
return this.each(function() {
var self = this
this.nodeType === ELEMENT_NODE && name.split(spaceRE).forEach(function(attribute) {
self.removeAttribute(attribute)
})
})
},
/**
* ## Lite.prototype.remove
*
* 删除节点。
*/
remove: function() {
return this.each(function() {
var parentNode = this.parentNode
parentNode && parentNode.removeChild(this)
})
},
/**
* ## Lite.prototype.appendTo
*
* 将html插入到Dom节点内底部。
*/
appendTo: function(target) {
var dom = [],
self = this
target.each(function() {
var node = this
self.each(function() {
dom.push(node.appendChild(this))
})
})
return $(dom)
},
/**
* ## Lite.prototype.after
*
* 在Dom节点之后插入html。
*/
after: function(html) {
return this.each(function() {
this.insertAdjacentHTML('afterend', html)
})
},
/**
* ## Lite.prototype.before
*
* 在Dom节点之前插入html。
*/
before: function(html) {
return this.each(function() {
this.insertAdjacentHTML('beforebegin', html)
})
},
/**
* ## Lite.prototype.append
*
* 在Dom节点内底部插入html。
*/
append: function(html) {
return this.each(function() {
this.insertAdjacentHTML('beforeend', html)
})
},
/**
* ## Lite.prototype.prepend
*
* 在Dom节点内头部插入html。
*/
prepend: function(html) {
return this.each(function() {
this.insertAdjacentHTML('afterbegin', html)
})
},
/**
* ## Lite.prototype.html
*
* 设置或获取Dom html。
*
*/
html: function(html) {
return html != _undefined ?
this.each(function() {
this.innerHTML = html
}) :
(this[0] ? this[0].innerHTML : null)
},
/**
* ## Lite.prototype.text
* 设置或获取Dom文本内容。
*/
text: function(text) {
return text != _undefined ?
this.each(function() {
this.textContent = text
}) :
(this[0] ? this[0].textContent : null)
}
}
}).call(Lite)
module.exports = _window.Zepto || Lite
<file_sep>/**
* # Loader
*
* 加载等待框。
*
*/
/**
* @require [core](./core.js.html)
* @extend [Dialog](./dialog.js.html)
*/
var core = require('./core'),
Dialog = require('./dialog')
/**
* ## Loader Constructor
*
* 继承于`Dialog`,可使用`Dialog`类中的方法。
*
* 使用方法:
*
* ```js
* var loader = new Kub.Loader()
*
* //隐藏loader
* loader.hide()
*
* var loader = new Kub.Loader({
* message: '定制提示内容'
* })
*
* ```
*/
function Loader(options) {
var opts = this.options = core.extend({}, _prototype.defaults, options || {}, {
showHeader: false,
buttons: null
})
Dialog.call(this, opts)
}
var _prototype = Loader.prototype = Object.create(Dialog.prototype)
_prototype.constructor = Loader
/**
* ## Loader.prototype.defaults
*
* `Loader` 默认配置项。
*
* 配置项说明:
*
* * `className`: `String` 弹窗类名,修改时需加上`kub-loader`默认类名。
*
* * `message` : `String` 提示内容。
*
* * `modal` : `Boolean` 是否显示遮罩层。
*/
_prototype.defaults = {
className: 'kub-loader',
modal: true,
message: '加载中…'
}
module.exports = Loader
<file_sep>/**
* # Toast
*
* 提示框
*/
/**
* @require [core](./core.js.html)
* @extend [Dialog](./dialog.js.html)
*/
var core = require('./core'),
Dialog = require('./dialog')
/**
* ## Toast Constructor
*
* 继承于`Dialog`,可使用`Dialog`类中的方法。
*
* 使用方法:
* ```js
* var toast = new Kub.Toast({
* message:'操作成功。'
* })
* ```
*/
function Toast(options){
var self = this,
opts = this.options = core.extend({}, _prototype.defaults, options||{},{
showHeader:false,
buttons:null
})
Dialog.call(this, opts)
//自动关闭
setTimeout(function(){
self.close()
}, opts.delay)
}
var _prototype = Toast.prototype = Object.create(Dialog.prototype)
_prototype.constructor = Toast
/**
* ## Toast.prototype.defaults
*
* `Toast`默认配置项。
*
* 配置项说明:
*
* * `message` : `String` 提示内容。
*
* * `className` : `String` 弹窗类名,修改时需加上`kub-toast`默认类名。
*
* * `top` : `Number` 距离顶部高度。
*
* * `delay` : `Number` 延迟时间。
*
* * `modal` : `Boolean` 是否显示遮罩层。
*/
_prototype.defaults = {
message:'',
className:'kub-toast',
top:50,
delay:2000,
modal:false
}
_prototype.setPosition = function(){
var top = this.options.top
this.$element.css({
top:core.isNumber(top) ? top + 'px' : top
})
return this
}
module.exports = Toast
<file_sep># 更新日志
## V2.4.0
### 新增功能
0. `lazyload` 组件新增校验是否支持webp格式方法`lazyload.prototype.isSoupportWebp`;
1. `lazyload` 组件初始化入参新增`imgToWebp`配置项,是否开启webp格式;
### 解决的bug
0. 修复`Datepicker`组件 && `swiper`组件在andriod7.0机型上面时间轴浮层滚动透传的问题;
1. `Datepicker`界面样式渐变属性`linear-gradient`写法向上兼容;
## v2.3.0
### 新增功能
0. 新增 `Popup` 组件;
1. 将 `Datepicker` 界面从 `Dialog` 换成 `Popup`;
## v2.2.0
### 解决的bug
0. 修复 `Datepicker.prototype.getDate` 方法返回多余时间区间问题;
1. 修复 `Dialog` 代码放到 `head` 区域时,无法插入节点;
2. 修复 `Lite.prototype.html` 无法插入 0 问题;
3. 修复 `Lite.prototype.text` 无法插入 0 问题;
4. 修复 `LazyLoad.prototype.isVisible` 对不可见元素检测失败问题;
### 新增功能
0. `LazyLoad` 组件增加`load`加载事件;
## v2.1.0
### 解决的bug
0. 修复`core.setQuerystring`在获取中文参数编码错误问题;
1. 修复`LazyLoad.prototype.load` 链式调用问题;
2. 修复`Lite`事件代理`this`指针指向问题;
3. 修复`Lite`事件代理不允许代理自身问题;
4. 修复`Lite.prototype.remove`无法移除文本节点问题;
### 优化点
0. 将兼容性解决代码抽离到`polyfill.js`中;
### 新增功能
0. 增加`Touch`移动事件组件;
1. 增加`Kub.version`版本号;
2. `Kub.Swiper`增加`auto`自动滚动功能;
## v2.0.3
### 解决的bug
0. 修复`Datepicker`键盘会弹出问题;
1. 修复`core.setQuerystring`会生成不符合规范的`a&a=1`地址;
## v2.0.2
### 解决的bug
0. 修复`Lite.prototype.text`问题;
## v2.0.1
### 解决的bug
0. 修复`swiper`组件切换失效问题;
1. 修复`core.extend`递归问题;
### 优化点
0. 去除`zepto`、`underscore`、`hammerjs`依赖;
1. 去除多余组件;
2. 去除多余不常用的API;
3. 将`os`模块抽离出`core`模块;
4. 增加`Lite`模块;
5. 增加嵌入样式到js中;
6. `Loader`组件增加可配置文案功能;
7. `setQuerystring`方法中`append`默认设置为`true`;
8. 增加样式可配置功能;
<file_sep>/**
* # DatePicker
*
* 时间选择器。格式化参照 [`DateHelper`](./date.js.html)
*
*/
/**
* @require [core](./core.js.html)
* @require [Lite](./lite.js.html)
* @require [detect](./detect.js.html)
* @require [Popup](./popup.js.html)
* @require [DateHelper](./date.js.html)
*/
var core = require('./core'),
$ = require('./lite'),
os = require('./detect'),
Popup = require('./popup'),
template = require('./tpl/datepicker');
require('./date')
var HEIGHT_UNIT = 54,
DURATION = 200,
COLUMN_SELECTOR = '.kub-datepicker-column',
POPUP_CLASS_NAME = 'kub-datepicker-popup',
DIALOG_BUTTON_SELECTOR = '.J_dialogButton',
COLUMN_ITEM_SELECTOR = 'li',
COLUMN_CONTAINER_SELECTOR = 'ul'
var $document = $(document),
isTouch = os.mobile
var START_EVENT = isTouch ? 'touchstart' : 'mousedown',
MOVE_EVENT = isTouch ? 'touchmove' : 'mousemove',
END_EVENT = isTouch ? 'touchend' : 'mouseup',
EVENT_NAME = 'click'
//渲染对话框
var render = function(datepicker) {
var options = datepicker.options,
html = template(options)
var popup = datepicker.$element[0].popup = new Popup({
title: options.title,
message: html,
className: options.className || '' + ' ' + POPUP_CLASS_NAME,
closeHandler:function(){
this.hide()
return false
}
})
var handlers = [
function(e) {
var cancel = options.cancel
cancel ? cancel.call(this, e, datepicker) : popup.hide()
},
function(e) {
var confirm = options.confirm,
formatDate = datepicker.getDate().format(options.format)
confirm ? confirm.call(this, e, datepicker, formatDate) : function() {
datepicker.$element[0].value = formatDate
popup.hide()
}()
}
]
//注册按钮事件
popup.$element.find(DIALOG_BUTTON_SELECTOR).on(EVENT_NAME, function(e) {
var index = parseInt($(this).attr('data-index')),
handler = handlers[index]
handler && handler.call(this, e, popup)
})
}
/**
* ## DatePicker Constructor
*
* `DatePicker` 构造函数。
*
* 使用:
* ```js
* //采用默认的format yyyy-MM-dd
* var datepicker = new Kub.DatePicker($('#J_datepicker'))
*
* //采用默认的format yyyy-MM-dd
* //可配置title 与 本地化
* var datepicker1 = new Kub.DatePicker($('#J_datepicker1'),{
* title:'Date Picker',
* locale:'en'
* })
*
* //自定义format
* var datepicker2 = new Kub.DatePicker($('#J_datepicker2'),{
* title:'选择时间',
* format:'yyyy-MM-dd,HH:mm:ss',
* confirm:function(e,datepicker,formatDate){
* //格式化后的 formatDate
* //手动关闭选择器
* datepicker.hide()
* }
* })
* ```
*/
var _prototype
//获取触摸点
var getCoords = function(event) {
var touches = event.touches,
data = touches && touches.length ? touches : event.changedTouches
return {
x: isTouch ? data[0].pageX : event.pageX,
y: isTouch ? data[0].pageY : event.pageY
}
}
//获取偏移
var getDistance = function(event, startCoords) {
var coords = getCoords(event),
x = coords.x - startCoords.x,
y = coords.y - startCoords.y
return {
distanceX: x,
distanceY: y
}
}
var returnFalse = function() {
return false
}
//获取当月最大天数
var getDays = function(year, month) {
return new Date(year, month + 1, 0).getDate()
}
//根据偏移量算出索引值
var getIndexByDistance = function(y, max) {
//去掉空白的两行
max = max - 5
y = y > 0 ? 0 : y
var index = Math.round(Math.abs(y) / HEIGHT_UNIT)
return index > max ? max : index
}
//设置偏移速度
var setDuration = function($this, duration) {
var $container = $this[0].$container,
transition = 'transform ' + duration + 'ms ease-out',
webkiTransition = '-webkit-transform ' + duration + 'ms ease-out'
$container.css({
'-webkit-transition': duration != null ? webkiTransition : duration,
'transition': duration != null ? transition : duration
})
}
//设置偏移速度
var setTranslate = function($this, x, y) {
var $container = $this[0].$container,
t
core.isNumber(x) && (x += 'px')
core.isNumber(y) && (y += 'px')
t = 'translate3d(' + x + ',' + y + ',0)'
!$container && ($container = $this[0].$container = $this.find(COLUMN_CONTAINER_SELECTOR))
$container.css({
'-webkit-transform': t,
'transform': t
})
}
//设置时间选择器中某一列的值,可设置年、月、日、时、分、秒的值
var setValue = function(datepicker, name, value) {
var $this = datepicker._ui[name],
index, $item
if ($this && ($item = $this.find(COLUMN_ITEM_SELECTOR + '[data-value="' + value + '"]')).length) {
index = parseInt($item.attr('data-index'))
$this[0].index = index
setTranslate($this, 0, -index * HEIGHT_UNIT)
}
}
//获取时间选择器中某一列的值,可获取年、月、日、时、分、秒的值
var getValue = function(datepicker, name) {
var $this = datepicker._ui[name],
$items, index, value
if ($this) {
$items = $this.find(COLUMN_ITEM_SELECTOR)
index = $this[0].index+2
value = parseInt($items.eq(index).attr('data-value'))
}
return value ? value : 0
}
//移除不需要的列
var removeColumns = function(format, ui) {
format.indexOf('y') === -1 && (ui.year.remove(), ui.year = null)
format.indexOf('M') === -1 && (ui.month.remove(), ui.month = null)
format.indexOf('d') === -1 && (ui.day.remove(), ui.day = null)
format.indexOf('H') === -1 && (ui.hour.remove(), ui.hour = null)
format.indexOf('m') === -1 && (ui.minute.remove(), ui.minute = null)
format.indexOf('s') === -1 && (ui.second.remove(), ui.second = null)
}
//重置每月最大天数
var setActualDays = function(datepicker, year, month) {
var days = getDays(year, month),
day = getValue(datepicker, 'day')
days < day && setValue(datepicker, 'day', days)
}
//绑定输入框聚焦事件
var bindInputFocusEvent = function(datepicker) {
datepicker.$element.on(EVENT_NAME, function(event) {
//使输入框失去焦点
datepicker.$element[0].blur()
datepicker.show()
event.preventDefault()
})
}
//绑定事件
var bindEvents = function(datepicker) {
var flag = false,
$activeElement,
$element = datepicker.$element[0].popup.$element
var start = function(event) {
flag = true
event = event.originalEvent || event
this.startCoords = getCoords(event)
$activeElement = $(this)
setDuration($activeElement, null)
},
move = function(event) {
if (!flag) return
event = event.originalEvent || event
var distance = getDistance(event, $activeElement[0].startCoords)
setTranslate($activeElement, 0, distance.distanceY - HEIGHT_UNIT * $activeElement[0].index)
event.preventDefault()
},
end = function(event) {
if (!flag) return
flag = false
event = event.originalEvent || event
var distance = getDistance(event, $activeElement[0].startCoords),
max = $activeElement.find(COLUMN_ITEM_SELECTOR).length,
index = getIndexByDistance(distance.distanceY - HEIGHT_UNIT * $activeElement[0].index, max)
$activeElement[0].index = Math.abs(index)
//验证是否存在31,30,29天
setActualDays(datepicker, getValue(datepicker, 'year'), getValue(datepicker, 'month'))
setDuration($activeElement, DURATION)
setTranslate($activeElement, 0, -HEIGHT_UNIT * $activeElement[0].index)
}
datepicker._ui.columns.each(function() {
$(this).on(START_EVENT, function() {
start.apply(this, arguments)
})
this.onselectstart = returnFalse
this.ondragstart = returnFalse
})
$element.on(MOVE_EVENT, move)
$element.on(END_EVENT, end)
bindInputFocusEvent(datepicker)
}
function init(datepicker) {
var options = datepicker.options,
$element,
ui
//创建对话框
render(datepicker)
$element = datepicker.$element[0].popup.$element
//缓存dom
ui = datepicker._ui = {
year: $element.find('.year'),
month: $element.find('.month'),
day: $element.find('.day'),
hour: $element.find('.hour'),
minute: $element.find('.minute'),
second: $element.find('.second')
}
ui.columns = $element.find(COLUMN_SELECTOR)
//设置块高度
HEIGHT_UNIT = ui.columns.find(COLUMN_ITEM_SELECTOR)[0].offsetHeight || HEIGHT_UNIT
//隐藏对话框
datepicker.hide()
//移除
removeColumns(options.format, ui)
//设置本地化
$element.addClass('kub-datepicker-' + options.locale)
//设置默认时间
datepicker.setDate(options.date)
//绑定事件
bindEvents(datepicker)
}
function DatePicker(element, options) {
this.$element = $(element)
this.options = core.extend({}, _prototype.defaults, options || {})
init(this)
}
_prototype = DatePicker.prototype
/**
* ## DatePicker.prototype.defaults
*
* 默认配置项
*
* 配置项说明:
*
* * `locale`: `String` 本地化。本地化采用CSS实现。
*
* * `title`: `String` 时间选择器弹窗名称。
*
* * `confirmText`: `String` 确认按钮名称。
*
* * `confirm`: `Function` 单击确认按钮时触发的事件。
*
* > 如果未传递,单击时会默认关闭弹窗,并对输入框赋值。
* >
* > 如果传递,需调用`datepicker.close()`手动关闭弹窗,并且需要对输入框赋值。
*
* * `cancelText`: `String` 取消按钮名称。
*
* * `cancel`: `Function` 单击取消按钮时触发的事件。
*
* > 如果未传递,单击时会默认关闭弹窗。
* >
* > 如果传递,需调用`datepicker.close()`关闭弹窗。
*
* * `format`: `String` 日期格式。
*
* * `className`: `String` 弹窗类名,修改时需加上`kub-datepicker-popup`默认类名。
*
* * `date`: `Date` 默认显示时间。
*
* * `yearRange`: `Array` 年份显示区间。
*/
_prototype.defaults = {
locale: 'zh',
title: '',
confirmText: '确定',
confirm: null,
cancelText: '取消',
cancel: null,
format: 'yyyy-MM-dd',
className: '',
date: new Date(),
yearRange: [1970, 2050]
}
/**
* ## DatePicker.prototype.setDate
*
* 设置时间选择器时间。
*
* @param {Date} date 时间
* @return {instance} 当前实例
*/
_prototype.setDate = function(date) {
var self = this,
year = date.getFullYear(),
month = date.getMonth()
setValue(self, 'year', year)
setValue(self, 'month', month)
setValue(self, 'day', date.getDate())
setValue(self, 'hour', date.getHours())
setValue(self, 'minute', date.getMinutes())
setValue(self, 'second', date.getSeconds())
return self
}
/**
* ## DatePicker.prototype.getDate
*
* 获取时间选择器选择的时间。
*
* @return {Date} 获取到的时间
*/
_prototype.getDate = function() {
var values = {
year: getValue(this, 'year'),
month: getValue(this, 'month'),
day: getValue(this, 'day'),
hour: getValue(this, 'hour'),
minute: getValue(this, 'minute'),
second: getValue(this, 'second')
}
return new Date(values.year, values.month, values.day, values.hour, values.minute, values.second)
}
/**
* ## DatePicker.prototype.close
*
* 关闭时间选择器。
*
* @return {instance} 当前实例
*/
_prototype.close = function() {
this.$element[0].popup.close()
return this
}
/**
* ## DatePicker.prototype.show
*
* 显示时间选择器。
*
* @return {instance} 当前实例
*/
_prototype.show = function() {
this.$element[0].popup.show()
return this
}
/**
* ## DatePicker.prototype.hide
*
* 隐藏时间选择器。
*
* @return {instance} 当前实例
*/
_prototype.hide = function() {
this.$element[0].popup.hide()
return this
}
module.exports = DatePicker
<file_sep>/**
* # Swiper
*
* 切换组件
*/
/**
* @require [core](./core.js.html)
* @require [os](./detect.js.html)
* @require [Lite](./lite.js.html)
*/
var core = require('./core'),
os = require('./detect'),
$ = require('./lite')
/**
* ## Swiper Constructor
*
* `Swiper`类。
*
* 使用方法:
* ```js
* new Kub.Swiper('.swiper',{
* slideSelector:'.slide',
* slideActiveClass:'active',
* paginationSelector:'.pagination li',
* paginationActiveClass:'pagination-active',
* slide:function(index){
* //当前滚动索引
* }
* })
* ```
*/
function Swiper(element, options) {
this.options = core.extend(true, {}, _prototype.defaults, options || {})
this.$element = $(element)
var ui = this._ui = {
slides: $(options.slideSelector),
paginations: $(options.paginationSelector)
}
ui.slidesLength = ui.slides.length
init(this)
}
var $document = $(document),
isTouch = os.mobile
var START_EVENT = isTouch ? 'touchstart' : 'mousedown',
MOVE_EVENT = isTouch ? 'touchmove' : 'mousemove',
END_EVENT = isTouch ? 'touchend' : 'mouseup',
RESIZE_EVENT = 'resize',
TRANSITIONEND_EVENT = 'transitionend',
WEBKIT_TRANSITIONEND_EVENT = 'webkitTransitionEnd',
HORIZONTAL = 'horizontal',
//粘性
VISCOSITY = 5,
//触摸点偏移量
TOUCH_THRESHOLD = 5
var _window = window,
_prototype = Swiper.prototype
//获取触摸点
var getCoords = function(event) {
var touches = event.touches,
data = touches && touches.length ? touches : event.changedTouches
return {
x: isTouch ? data[0].pageX : event.pageX,
y: isTouch ? data[0].pageY : event.pageY
}
}
//获取偏移
var getDistance = function(event, startCoords) {
var coords = getCoords(event),
x = coords.x - startCoords.x,
y = coords.y - startCoords.y
return {
distanceX: x,
distanceY: y
}
}
//获取位置与索引
var getCoordinates = function(swiper, distanceX, distanceY) {
var element = swiper._ui.slides[0],
w = element.offsetWidth,
h = element.offsetHeight,
l = swiper._ui.slidesLength,
index = swiper._ui.active,
active = index,
threshold = swiper.options.threshold,
reach,
_distanceY = Math.abs(distanceY),
_distanceX = Math.abs(distanceX)
if (swiper.options.direction === HORIZONTAL) {
//达到门槛
reach = threshold < _distanceX
//横向
if (distanceX > 0 && index == 0) {
//最左侧
distanceX = distanceX / VISCOSITY
index = 0
} else if (distanceX < 0 && index == l - 1) {
//最右侧
distanceX = distanceX / VISCOSITY
index = l - 1
} else if (reach) {
//达到最小偏移量
//取整
var s = Math.round(_distanceX / w)
s = s == 0 ? 1 : s
//向右或者向左
index = distanceX > 0 ? index - s : index + s
}
return {
x: distanceX + (-w * active),
y: 0,
index: index,
isDefaultPrevented: !(!reach && _distanceX < _distanceY && TOUCH_THRESHOLD < _distanceY)
}
} else {
//达到门槛
reach = threshold < _distanceY
//垂直
if (distanceY > 0 && index == 0) {
//最上
distanceY = distanceY / VISCOSITY
index = 0
} else if (distanceY < 0 && index == l - 1) {
//最下
distanceY = distanceY / VISCOSITY
index = l - 1
} else if (reach) {
//达到最小偏移
var s = Math.round(_distanceY / h)
s = s == 0 ? 1 : s
index = distanceY > 0 ? index - s : index + s
}
return {
x: 0,
y: distanceY + (-h * active),
index: index,
isDefaultPrevented: true
}
}
}
var returnFalse = function() {
return false
}
//添加重复子节点
var appendCloneChildren = function(swiper) {
if (swiper.options.infinite) {
var $slides = swiper._ui.slides,
first = $slides[0],
last = $slides[swiper._ui.slidesLength - 1],
parentElement = first.parentElement
parentElement.insertBefore(last.cloneNode(true), first)
parentElement.appendChild(first.cloneNode(true))
swiper._ui.slidesLength += 2
}
}
//重置索引值
var resetSlideIndex = function(swiper) {
var index = swiper._ui.active,
length = swiper._ui.slidesLength
if (swiper.options.infinite) {
if (index === length - 1) {
swiper.slide(1, 0)
}
if (index === 0) {
swiper.slide(length - 2, 0)
}
}
}
//设置偏移量
var setTranslate = function($element, x, y) {
core.isNumber(x) && (x += 'px')
core.isNumber(y) && (y += 'px')
var t = 'translate3d(' + x + ',' + y + ',0)'
$element.css({
'-webkit-transform': t,
'transform': t
})
}
//设置偏移速度
var setDuration = function($element, duration) {
core.isNumber(duration) && (duration += 'ms')
$element.css({
'-webkit-transition-duration': duration,
'transition-duration': duration
})
}
var getActualIndex = function(index, length) {
return index < 0 ? 0 : index >= length ? length - 1 : index
}
//设置容器偏移量
var setContainerTranslate = function(swiper, x, y, duration) {
var $element = swiper.$element
duration = duration || 0
setDuration($element, duration)
setTranslate($element, x, y)
}
//添加选中类
var setActiveClass = function(swiper, index) {
var options = swiper.options,
slideActiveClass = options.slideActiveClass,
paginationActiveClass = options.paginationActiveClass
//添加选中的class
swiper._ui.slides.removeClass(slideActiveClass).eq(index).addClass(slideActiveClass)
swiper._ui.paginations.removeClass(paginationActiveClass).eq(index).addClass(paginationActiveClass)
}
//监听slide完成事件
var bindTransitionEndEvent = function(swiper) {
var $element = swiper.$element
var handler = function() {
var options = swiper.options,
callback = options.slide,
index = swiper._ui.active
resetSlideIndex(swiper)
//计算出真实索引值
options.infinite && (index = swiper._ui.active - 1)
callback && callback.call(swiper, index)
}
//duration == 0 无法触发
//translate 值未改变也无法触发
$element.on(TRANSITIONEND_EVENT, handler).on(WEBKIT_TRANSITIONEND_EVENT, handler)
}
//监听横竖屏切换
var bindOrientationChangeEvent = function(swiper) {
var timer
function handler() {
timer && clearTimeout(timer)
timer = setTimeout(function() {
swiper.slide(swiper._ui.active)
}, 200)
}
$(_window).on(RESIZE_EVENT, handler)
}
//绑定事件
var bindEvents = function(swiper) {
var flag = false,
startCoords
var start = function(event) {
stopAuto(swiper)
flag = true
event = event.originalEvent || event
resetSlideIndex(swiper)
startCoords = getCoords(event)
setDuration(swiper.$element, null)
},
move = function(event) {
if (!flag) return
event = event.originalEvent || event
var distance = getDistance(event, startCoords),
coordinates = getCoordinates(swiper, distance.distanceX, distance.distanceY)
coordinates.isDefaultPrevented && (event.preventDefault(),setTranslate(swiper.$element, coordinates.x, coordinates.y))
},
end = function(event) {
if (!flag) return
flag = false
event = event.originalEvent || event
var distance = getDistance(event, startCoords),
index = getCoordinates(swiper, distance.distanceX, distance.distanceY).index
swiper.slide(index)
beginAuto(swiper)
}
//监听横竖屏
bindOrientationChangeEvent(swiper)
//触发回调函数
bindTransitionEndEvent(swiper)
swiper.$element.on(START_EVENT, start)
swiper.$element.on(MOVE_EVENT, move)
swiper.$element.on(END_EVENT, end)
swiper.$element[0].onselectstart = returnFalse
swiper.$element[0].ondragstart = returnFalse
}
//偏移到指定的位置
var slideTo = function(swiper, index, duration) {
var element = swiper._ui.slides[0]
//由于移动端浏览器 transition 动画不支持百分比,所以采用像素值
if (swiper.options.direction === HORIZONTAL) {
//横向
var w = element.offsetWidth
setContainerTranslate(swiper, -index * w, 0, duration)
} else {
//垂直
var h = element.offsetHeight
setContainerTranslate(swiper, 0, -index * h, duration)
}
}
// 开始自动切换
var beginAuto = function(swiper){
var options = swiper.options,
_ui = swiper._ui,
auto = options.auto
auto && (swiper._timer = setInterval(function(){
// 由于一些特殊设计
// 对非循环滚动采用自动计算索引值的方式
options.infinite ? swiper.next() : swiper.slide( (_ui.active + 1) % _ui.slidesLength )
}, auto))
}
// 停止自动切换
var stopAuto = function(swiper){
var timer = swiper._timer
timer && clearInterval(timer)
}
//初始化
var init = function(swiper) {
var options = swiper.options
appendCloneChildren(swiper)
//设置默认样式
swiper.$element.css(options.style.swiper)
var initialSlide = options.initialSlide || 0
options.infinite && (initialSlide = initialSlide + 1)
//滚动到默认位置
swiper.slide(initialSlide, 0)
beginAuto(swiper)
//绑定事件
bindEvents(swiper)
}
/**
* ## Swiper.prototype.defaults
*
* `Swiper`默认配置项。
*
* 配置项说明:
*
* * `direction`: `String` 切换方向。horizontal :横向, vertical :纵向。
*
* * `threshold`: `Number` 最小触发距离。手指移动距离必须超过`threshold`才允许切换。
*
* * `duration`: `Number` 切换速度。
*
* * `auto`: `Number` 自动切换速度。0表示不自动切换,默认为0 。
*
* * `infinite`: `Boolean` 是否循环滚动 true :循环 false :不循环。
*
* * `initialSlide`: `Number` 初始化滚动位置。
*
* * `slideSelector`: `Selector` 滚动元素。
*
* * `slideActiveClass`: `String` 滚动元素选中时的类名。
*
* * `paginationSelector`: `Selector` 缩略图或icon。
*
* * `paginationActiveClass`: `String` 缩略图或icon选中时的类名。
*
* * `slide`: `Function` 切换回调函数。
*/
_prototype.defaults = {
//vertical
direction: HORIZONTAL,
threshold: 50,
duration: 300,
infinite: false,
initialSlide: 0,
slideSelector: '',
slideActiveClass: '',
paginationSelector: '',
paginationActiveClass: '',
slide: null,
style: {
swiper: {
'-webkit-transition-property': '-webkit-transform',
'transition-property': 'transform',
'-webkit-transition-timing-function': 'ease-out',
'transition-timing-function': 'ease-out'
}
}
}
/**
* ## Swiper.prototype.slide
*
* 滚动到指定索引值位置
*
* @param {index} index 滚动索引值
* @param {duration} duration 滚动速度,默认配置的`duration`。
* @return {instance} 当前实例
*/
_prototype.slide = function(index, duration) {
var options = this.options
//如果speed为空,则取默认值
duration = duration == null ? options.duration : duration
//取出实际的索引值,保存当前索引值
this._ui.active = index = getActualIndex(index, this._ui.slidesLength)
//通过索引值设置偏移
slideTo(this, index, duration)
//设置选中状态Class
setActiveClass(this, options.infinite ? index - 1 : index)
return this
}
/**
* ## Swiper.prototype.next
*
* 切换到下一个
*
* @param {duration} duration 滚动速度,默认配置的`duration`。
* @return {instance} 当前实例
*/
_prototype.next = function(duration) {
return this.slide(this._ui.active + 1, duration)
}
/**
* ## Swiper.prototype.prev
*
* 切换到上一个
*
* @param {duration} duration 滚动速度,默认配置的`duration`。
* @return {instance} 当前实例
*/
_prototype.prev = function(duration) {
return this.slide(this._ui.active - 1, duration)
}
module.exports = Swiper
<file_sep>var _window = window,
_document = document
var ELEMENT_NODE = 1,
ELEMENT_PROTOTYPE = Element.prototype
//CustomEvent polyfill
//android 4.3
if (!_window.CustomEvent) {
var CustomEvent = function(event, params) {
var evt
params = params || {
bubbles: false,
cancelable: false,
detail: undefined
}
evt = _document.createEvent("CustomEvent")
evt.initCustomEvent(event, params.bubbles, params.cancelable, params.detail)
return evt
}
CustomEvent.prototype = _window.Event.prototype
_window.CustomEvent = CustomEvent
}
//Element.prototype.matches polyfill
if (typeof ELEMENT_PROTOTYPE.matches !== 'function') {
ELEMENT_PROTOTYPE.matches = ELEMENT_PROTOTYPE.msMatchesSelector || ELEMENT_PROTOTYPE.mozMatchesSelector || ELEMENT_PROTOTYPE.webkitMatchesSelector || function matches(selector) {
var element = this,
// 查找当前节点的根节点时,必须使用element.ownerDocument
// 当节点插入到iframe中时,该节点的document就不是父窗口中的document,而是iframe中的document
// 会造成 document.querySelectorAll(selector) 查询不到改节点,所以需要element.ownerDocument替代document
elements = element.ownerDocument.querySelectorAll(selector),
index = 0
while (elements[index] && elements[index] !== element) {
++index
}
return !!elements[index]
}
}
//Element.prototype.closest polyfill
if (typeof ELEMENT_PROTOTYPE.closest !== 'function') {
ELEMENT_PROTOTYPE.closest = function closest(selector) {
var element = this
while (element && element.nodeType === ELEMENT_NODE) {
if (element.matches(selector)) {
return element
}
element = element.parentNode
}
return null
}
}
<file_sep>/**
* # os
*
* 检测系统类型与版本,包含系统类型与版本信息。
*
* 只检测Android 与 IOS, window phone(window phone 未进行完全测试)
*
* `os` 返回以下相关属性:
*
* `android` :是否是Android
*
* `ios` :是否是IOS系统
*
* `ipad` :是否是iPad
*
* `iphone` :是否是iPhone
*
* `ipod` :是否是iPod
*
* `mobile` :是否是移动端
*
* `phone` :是否是手机
*
* `tablet` :是否是平板
*
* `version` :系统版本
*
* `wp` : 是否是window phone
*
*/
var ua = navigator.userAgent,
android = ua.match(/(Android);?[\s\/]+([\d.]+)?/),
ipad = ua.match(/(iPad).*OS\s([\d_]+)/),
ipod = ua.match(/(iPod)(.*OS\s([\d_]+))?/),
iphone = !ipad && ua.match(/(iPhone\sOS)\s([\d_]+)/),
wp = ua.match(/Windows Phone ([\d.]+)/),
os = {}
//android
android ? (os.android = true, os.version = android[2]) : (os.android = false)
//iphone
iphone && !ipod ? ( os.ios = os.iphone = true, os.version = iphone[2].replace(/_/g, '.') ) : (os.iphone = false)
//ipad
ipad ? ( os.ios = os.ipad = true, os.version = ipad[2].replace(/_/g, '.') ) : (os.ipad = false)
//ipod
ipod ? ( os.ios = os.ipod = true, os.version = ipod[3].replace(/_/g, '.') ) : (os.ipod = false)
//window phone
wp ? ( os.wp = true, os.version = wp[1]) : (os.wp = false)
//ios
!os.iphone && !os.ipad && !os.ipod && (os.ios = false)
//手机
os.phone = os.android && /mobile/i.test(ua) || os.iphone || os.wp ? true : false
//平板
os.tablet = !os.phone && ( os.android || os.ipad || /window/i.test(ua) && /touch/i.test(ua) ) ? true : false
//移动端
os.mobile = os.phone || os.tablet
module.exports = os
<file_sep>module.exports = function(data){
var __t,__p='',__j=Array.prototype.join,print=function(){__p+=__j.call(arguments,'');};
__p+=''+
((__t=(data.message))==null?'':__t)+
' <div class="kub-prompt-input-wrapper"><input placeholder="'+
((__t=( data.placeholder))==null?'':__t)+
'" type="'+
((__t=( data.inputType))==null?'':__t)+
'" value="'+
((__t=( data.defaultValue))==null?'':__t)+
'" class="kub-prompt-input J_input"></div>';
return __p;
};<file_sep>/**
* # Kub
*
* 与`Kub`对象一致,增加样式文件。
*
*/
require('../less/kub.less')
module.exports = require('./kub')
<file_sep>var Kub = window.Kub;
var core = Kub.core;
describe("core module:", function() {
xdescribe("os", function(){});
describe("core.extend()", function(){
var target, source, source1, sourceWithProto;
beforeEach(function(){
target = {
samename: "fisrtname",
uniquename: "uniquename"
};
source = {
samename: "secondname",
extendArr: [3, 4],
extendObj: {
a: 1,
b: [1, 2]
},
};
source1 = {
extendName: "source1",
extendArr: [1, 2]
};
function F(){
this.instanceProperty = "instanceProperty";
}
F.prototype.protoProperty = "protoProperty";
sourceWithProto = new F();
});
it("should support basic copy and rewrite if conflict", function(){
var res = core.extend(target, source);
expect(res.samename).toBe(source.samename);
expect(res.extendArr).toBe(source.extendArr);
expect(res.extendObj).toBe(source.extendObj);
});
xit("should support deep clone", function(){
var res = core.extend(true, target, source);
expect(res.extend).not.toBe(source.extend);
});
it("should have side effect with the target parameter", function(){
var res = core.extend(target, source);
expect(target).toBe(res);
});
it("does not work with prototype property, only with own perperty", function(){
var res = core.extend(target, sourceWithProto);
expect(res.instanceProperty).toBe(sourceWithProto.instanceProperty);
expect(res.protoProperty).toBeUndefined();
});
});
});
<file_sep># 轻量级移动端组件库 Kub
## 设计
0. Kub 由多个组件构成,组件之间依赖较低;
1. 组件尽量做到轻量且扩展性良好;
2. 组件对外提供稳定的API,在使用时可通过参数配置或者继承实现定制化的组件;
3. 组件采用`commonjs`规范,采用`webpack`编译,对外提供源文件与编译后的文件;
4. 提供模板源文件与预编译后的文件;
5. `Kub`对外提供暴露全局的`Kub`变量,所有组件绑定在该变量中;
6. 样式采用`less`编写,对外提供源文件与编译后的文件;
7. 样式可统一在`variables.less`文件中配置;
8. 对外提供不包含样式的`kub.js`以及包含样式的`kub.style.js`;
## 兼容性
0. ios7及以上版本;
1. Android4.0及以上版本;
## 版本
0. 遵循[语义化版本规范](http://semver.org/lang/zh-CN/);
1. `master`分支为最新的稳定的发布版本;
2. `tags`中的分支对应每一个发布版本;
3. 其余分支为开发分支,为不稳定版本;
4. `1.*` 版本将不再升级与维护,除非有重大bug;
## 安装
### CDN
```
<link rel="stylesheet" type="text/css" href="//assets.geilicdn.com/v-components/kubjs/2.4.0/index.css">
<script type="text/javascript" src="//assets.geilicdn.com/v-components/kubjs/2.4.0/index.js"></script>
<script type="text/javascript" src="//assets.geilicdn.com/v-components/kubjs/2.4.0/index.style.js"></script>
```
### git
```
git clone https://github.com/longjiarun/kubjs.git kub
```
### npm
```
npm install kub --save
//指定版本
npm install kub@version --save
```
### bower
```
bower install kub --save
//指定版本
bower install kub#version --save
```
## 使用
### 使用编译后的文件`kub.js`及`kub.style.js`。
1、如果组件名称首字母大小,则暴露的是类,需实例化;
```
//组件为类
var dialog = new Kub.Dialog({
//配置参数
});
```
2、如果组件名称首字母小写,则暴露的是对象或函数,无需实例化;
```
//组件为实例化后的对象
Kub.dateHelper.format(new Date(),'yyyy-MM-dd')
//组件为函数
Kub.cookie('name','kub');
//组件为对象
Kub.os.ios
```
### 使用源文件。
1、bower
```
//引用kub.js
var Kub = require('../../bower_components/kub/src/js/kub')
new Kub.Dialog()
//引用单个组件
var Dialog = require('../../bower_components/kub/src/js/dialog')
new Dialog()
//使用less
require('../../bower_components/kub/src/less/dialog.less')
```
2、npm
```
//引用kub.js
var Kub = require('kub')
new Kub.Dialog()
//引用单个组件
var Dialog = require('kub/src/js/dialog')
new Dialog()
//使用less
require('kub/src/less/dialog.less')
```
## 与V1版本的区别
描述与版本V1的区别,方便迁移到版本V2。
0. 模块规范由`umd`修改为`commonjs`;
1. 去除`zepto`、`underscore`、`hammerjs`依赖;
2. 去除`js/extend`目录中的组件;
3. 去除`calculator`组件;
4. 将`os`模块迁移到`detect`中,绑定由`Kub.core.os`迁移到`Kub.os`;
5. 增加`kub.js`与`kub.style.js`文件;
6. 将模板从组件中抽离,提供模板预编译文件;
7. 去除多余API,详见API文档;
8. 将`css`文件夹名称修改为`less`;
9. 将样式可配置项统一配置在`variables.less`中;
10. 在`variables.less`中提供单位可配置变量`@unit: 1px;`;
## 问题
0. 通过[Github issues](https://github.com/longjiarun/kubjs/issues)反馈;
1. 通过[Email](mailto:<EMAIL>)反馈;
## API文档
[查看文档](http://h5.weidian.com/v-components/kubjs/docs/v2.4.0/kub.js.html)
<file_sep>/**
* # DateHelper
*
* 日期格式化组件。
*
* 格式化字符:
*
yyyy : 四位年。例如:2015
yy : 二位年份,最后两位。例如 15
MMMM : 全称月份。例如 January
MMM : 简称月份。例如 Jan
MM : 两位月份,小于10显示03。例如:11
M : 一位月份,小于10显示3.例如:3或者11
dddd : 全称星期。例如:星期一,Sunday
ddd : 简称星期。例如:一,Sun
dd : 两位天数。类似于MM
d : 一位天数。类似于M
HH : 两位小时。类似于MM
H : 一位小时。类似于M
mm : 两位分钟。类似于MM
m : 一位分钟。类似于M
ss : 两位秒数。类似于MM
s : 一位秒数。类似于M
aa : 全称上午或者下午。例如A.M.,P.M.
a : 简称上午或者下午。例如AM.
*/
/**
* ## DateHelper Constructor
*
* `DateHelper` 模块,对外提供的是实例化的对象。
*
* 使用:
* ```js
* //String to Date
*
* '2015-05-20'.parseDate('yyyy-MM-dd')
*
* //格式化日期
* new Date().format('yyyy-MM-dd,HH:mm:ss')
*
* ```
*/
function DateHelper() {
}
var _prototype = DateHelper.prototype
var get2Year = function(date) {
return (date.getFullYear() + '').replace(/\d{2}$/, '00') - 0
}
var get2 = function(value) {
return value < 10 ? '0' + value : value
}
var getAmPm = function(date) {
return date.getHours() < 12 ? 0 : 1
}
//获取相对应的日期相关数据
var getValueByPattern = function(datehelper, fmt, date) {
var patterns = {
yyyy: date.getFullYear(),
yy: date.getFullYear() - get2Year(date),
MMMM: datehelper.i18n[datehelper.locale].month.full[date.getMonth()],
MMM: datehelper.i18n[datehelper.locale].month.abbr[date.getMonth()],
MM: get2(date.getMonth() + 1),
M: date.getMonth() + 1,
dddd: datehelper.i18n[datehelper.locale].day.full[date.getDay()],
ddd: datehelper.i18n[datehelper.locale].day.abbr[date.getDay()],
dd: get2(date.getDate()),
d: date.getDate(),
HH: get2(date.getHours()),
H: date.getHours(),
mm: get2(date.getMinutes()),
m: date.getMinutes(),
ss: get2(date.getSeconds()),
s: date.getSeconds(),
aa: datehelper.i18n[datehelper.locale].amPm.full[getAmPm(date)],
a: datehelper.i18n[datehelper.locale].amPm.abbr[getAmPm(date)]
}
return patterns[fmt]
}
//本地化,目前包含`en`与`zh`
_prototype.i18n = {
en: {
month: {
abbr: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'],
full: ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December']
},
day: {
abbr: ['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat'],
full: ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']
},
amPm: {
abbr: ['AM', 'PM'],
full: ['A.M.', 'P.M.']
}
},
zh: {
month: {
abbr: ['一月', '二月', '三月', '四月', '五月', '六月', '七月', '八月', '九月', '十月', '十一月', '十二月'],
full: ['一月份', '二月份', '三月份', '四月份', '五月份', '六月份', '七月份', '八月份', '九月份', '十月份', '十一月份', '十二月份']
},
day: {
abbr: ['日', '一', '二', '三', '四', '五', '六'],
full: ['星期日', '星期一', '星期二', '星期三', '星期四', '星期五', '星期六']
},
amPm: {
abbr: ['上午', '下午'],
full: ['上午', '下午']
}
}
}
//默认中文
_prototype.locale = 'zh'
/**
* ## DateHelper.prototype.addLocale
*
* 添加本地化
*
* @param {String} name 本地化名称
* @param {Object} locale 本地化数据
* @return {instance} 当前实例
*/
_prototype.addLocale = function(name, locale) {
name && locale && (this.i18n[name] = locale)
return this
}
/**
* ## DateHelper.prototype.setLocale
*
* 设置默认本地化
*
* @param {String} name 本地化名称
* @return {instance} 当前实例
*/
_prototype.setLocale = function(name) {
this.locale = name
return this
}
/**
* ## DateHelper.prototype.format
*
* 格式化日期。
*
* @param {Date} date 日期
* @param {String} format 日期格式
* @return {String} 格式化以后的日期
*/
_prototype.format = function(date, format) {
var self = this
if (!date) return
format = format || 'yyyy-MM-dd'
format = format.replace(/(yyyy|yy|MMMM|MMM|MM|M|dddd|ddd|dd|d|HH|H|mm|m|ss|s|aa|a)/g, function(part) {
return getValueByPattern(self, part, date)
})
return format
}
/**
* ## DateHelper.prototype.parse
*
* 转换日期。
*
* 此方法存在一个BUG,例如:
*
* ```js
*
* //1112会被计算在MM内。
* dateHelper.parse('2015-1112','yyyy-MMdd')
*
*
* //所以在使用parse方法时,每一个串使用字符分隔开。类似于:
*
* dateHelper.parse('2015-11-12','yyyy-MM-dd')
*
* ```
*
* @param {String} input 字符串
* @param {String} format 格式化字符串
* @return {Date} 格式化的日期
*/
_prototype.parse = function(input, format) {
if (!input || !format) return
var parts = input.match(/(\d+)/g),
i = 0,
fmt = {}
// extract date-part indexes from the format
format.replace(/(yyyy|yy|MM|M|dd|d|HH|H|mm|m|ss|s)/g, function(part) {
fmt[part] = i++
})
var year = parts[fmt['yyyy']] || (parseInt(parts[fmt['yy']], 10) + get2Year(new Date())) || 0,
month = (parts[fmt['MM']] - 1) || (parts[fmt['M']] - 1) || 0,
day = parts[fmt['dd']] || parts[fmt['d']] || 0,
hour = parts[fmt['HH']] || parts[fmt['H']] || 0,
minute = parts[fmt['mm']] || parts[fmt['m']] || 0,
second = parts[fmt['ss']] || parts[fmt['s']] || 0
return new Date(year, month, day, hour, minute, second)
}
var dateHelper = new DateHelper()
// 将 parseDate 方法绑定在 `String` 原型上
String.prototype.parseDate = function(format) {
return dateHelper.parse(this, format)
}
// 将 format 方法绑定在 `Date` 原型上
Date.prototype.format = function(format) {
return dateHelper.format(this, format)
}
module.exports = dateHelper
<file_sep>/**
* # Prompt
*
* prompt 输入框。
*/
/**
* @require [core](./core.js.html)
* @extend [Dialog](./dialog.js.html)
*/
var core = require('./core'),
Dialog = require('./dialog'),
template = require('./tpl/prompt')
var INPUT_SELECTOR = '.J_input'
/**
* ## Prompt Constructor
*
* 继承于`Dialog`,可使用`Dialog`类中的方法。
*
* 使用方法:
* ```js
* var prompt = new Kub.Prompt({
* confirm:function(event,dialog,value){
* //输入框输入的值 value
* }
* })
* ```
*/
function Prompt(options) {
var opts = this.options = core.extend({}, _prototype.defaults, options || {})
opts.buttons = [{
text: opts.cancelText,
handler: opts.cancel || function(e, dialog) {
dialog.close()
}
}, {
text: opts.confirmText,
handler: function(e, dialog) {
var value = dialog.$element.find(INPUT_SELECTOR)[0].value
opts.confirm && opts.confirm.call(this, e, dialog, value)
}
}]
opts.message = template(opts)
Dialog.call(this, opts)
}
var _prototype = Prompt.prototype = Object.create(Dialog.prototype)
_prototype.constructor = Prompt
/**
* ## Prompt.prototype.defaults
*
* `Prompt`默认配置项。
*
* 配置项说明:
*
* * `confirmText` : `String` 确认按钮名称。
*
* * `confirm` : `Function` 单击确认按钮时触发的事件。用于用户单击确认按钮执行事件。需调用`dialog.close()`手动关闭弹窗。
*
* * `cancelText` : `String` 取消按钮名称。
*
* * `cancel` : `Function` 单击取消按钮时触发的事件。
*
* > 如果未传递,单击时会默认关闭弹窗。
* >
* > 如果传递,需调用 `dialog.close()`关闭弹窗。
*
* * `showHeader` : `Boolean` 是否显示头部。
*
* * `className` : `String` 弹窗类名,修改时需加上`kub-prompt`默认类名。
*
* * `modal` : `Boolean` 是否显示遮罩层。
*
* * `message` : `String` 提示内容。
*
* * `inputType` : `String` 输入框类型。
*
* * `placeholder` : `String` 输入框 `placeholder` 属性。
*
* * `defaultValue` : `String` 输入框默认值。
*/
_prototype.defaults = {
confirmText: '确定',
confirm: null,
cancelText: '取消',
cancel: null,
showHeader: false,
className: 'kub-prompt',
modal: true,
message:'',
inputType: 'text',
placeholder: '',
defaultValue: ''
}
module.exports = Prompt
<file_sep>/**
* # Touch
*
* 移动端事件组件。
*
* 支持的事件包含:
*
* `tap` `longtap`
*
* `panstart` `panmove` `panup` `pandown` `panleft` `panright` `panend`
*
* `swipeleft` `swiperight` `swipeup` `swipedown`
*
*/
/**
* @require [polyfill](./polyfill.js.html)
*/
require('./polyfill')
var MOBILE_REGEXP = /mobile|tablet|ip(ad|hone|od)|android/i
var _window = window,
isTouch = 'ontouchstart' in _window && MOBILE_REGEXP.test(navigator.userAgent)
var EVENTS_METHODS = [
'preventDefault',
'stopImmediatePropagation',
'stopPropagation'
]
var SWIPE_THRESHOLD = 10,
SWIPE_VELOCITY = 0.25,
SWIPE_MAX_MOVEMENT = 6,
TAP_TIMEOUT = 200,
TAP_THRESHOLD = 9,
LONGTAP_TIMEOUT = 500,
START_EVENT = isTouch ? 'touchstart' : 'mousedown',
MOVE_EVENT = isTouch ? 'touchmove' : 'mousemove',
END_EVENT = isTouch ? 'touchend' : 'mouseup',
DIRECTION_ANGLE = 25,
DIRECTIONS = ['up', 'right', 'down', 'left'],
SWIPE_EVENT = 'swipe',
PAN_EVENT = 'pan',
PAN_START_EVENT = 'panstart',
PAN_MOVE_EVENT = 'panmove',
PAN_END_EVENT = 'panend',
TAP_EVENT = 'tap',
LONGTAP_EVENT = 'longtap'
// 获取位移量
var distance = function(p1, p2) {
return Math.round(Math.sqrt(Math.pow((p1.x - p2.x), 2) + Math.pow((p1.y - p2.y), 2)))
}
// 获取角度,通过获取角度从而获取方向
var angle = function(p1, p2) {
var d = Math.abs(p2.x - p1.x)
return Math.round(Math.acos(d / Math.sqrt(Math.pow(d, 2) + Math.pow(p2.y - p1.y, 2))) * 57.3)
}
// 获取方向
var direction = function(p1, p2) {
return (angle(p1, p2) > DIRECTION_ANGLE) ? ((p1.y < p2.y) ? 2 : 0) : ((p1.x < p2.x) ? 1 : 3)
}
// 如果触摸点位移大于 SWIPE_THRESHOLD 而且速度大于 SWIPE_VELOCITY
var matchSwipe = function(threshold, interval) {
return threshold != null && threshold > SWIPE_THRESHOLD && threshold / interval > SWIPE_VELOCITY
}
// 如果触摸点位置大于 TAP_THRESHOLD 而且间隔时间小于 TAP_TIMEOUT
var matchTap = function(threshold, interval) {
return threshold != null && threshold < TAP_THRESHOLD && interval < TAP_TIMEOUT
}
// 获取触摸点数据
var getCoords = function(event) {
var touches = event.touches,
data = touches && touches.length ? touches : event.changedTouches
return {
x: isTouch ? data[0].clientX : event.clientX,
y: isTouch ? data[0].clientY : event.clientY,
e: isTouch ? data[0].target : event.target
}
}
// 获取事件位置数据
var getEventDetail = function(coords) {
return {
x: coords.x,
y: coords.y
}
}
// 获取偏移值与时间间隔
var getThresholdAndInterval = function(p1,p2){
return {
threshold : p1 && p2 && distance(p1, p2),
interval : p1 && p2 && (p2.t.getTime() - p1.t.getTime())
}
}
// 触发事件
var trigger = function(element, type, originalEvent, detail) {
var event = new _window.CustomEvent(type, {
detail: detail,
bubbles: true,
cancelable: true
})
// 存储原事件对象
event.originalEvent = originalEvent
EVENTS_METHODS.forEach(function(name){
event[name] = function(){
originalEvent[name].apply(originalEvent,arguments)
}
})
element && element.dispatchEvent && element.dispatchEvent(event)
}
var on = function(element, type, handler) {
element.addEventListener(type, handler, false)
}
var clearTime = function(timer) {
timer && clearTimeout(timer)
timer = null
}
var findMatchedDirection = function(actions){
var index = 0, max = actions[index]
actions.forEach(function(value,i){
value > max && (max = value, index = i)
})
return index
}
/**
* ## Touch Constructor
*
* `Touch`类。
*
* 使用方法:
* ```js
*
* new Kub.Touch(element)
*
* // swipeleft 事件,支持事件代理
* element.addEventListener('swipeleft','div',function(){
* //do something
* })
*
* // tap 事件
* element.addEventListener('tap',function(){
* //do something
* })
*
* ```
*/
function Touch(element) {
var moveFlag = false,
target,
p1,
p2,
longTapTimer,
cancelTap = false,
actions,
actionsLength
on(element, START_EVENT, function(event) {
var coords = getCoords(event)
p1 = coords
p1.t = new Date()
p2 = p1
actions = [0,0,0,0]
actionsLength = 0
cancelTap = false
target = coords.e
//触发 longtap 事件
isTouch && (longTapTimer = setTimeout(function() {
trigger(target, LONGTAP_EVENT, event)
}, LONGTAP_TIMEOUT))
})
on(element, MOVE_EVENT, function(event) {
if(!target){
return
}
var coords = getCoords(event),
detail = getEventDetail(coords),
thresholdAndInterval,
direct
p2 = coords
p2.t = new Date()
thresholdAndInterval = getThresholdAndInterval(p1,p2)
// 如果触摸点不符合 longtap 触发条件,则取消长按事件
if(!cancelTap && !matchTap(thresholdAndInterval.threshold, thresholdAndInterval.interval)){
clearTime(longTapTimer)
cancelTap = true
}
//触发 panstart 事件
!moveFlag && trigger(target, PAN_START_EVENT, event, detail)
direct = direction(p1, p2)
// 取出前SWIPE_MAX_MOVEMENT移动记录
actionsLength < SWIPE_MAX_MOVEMENT && (actions[direct] += 1, actionsLength += 1)
//触发 pan['up', 'right', 'down', 'left'] 事件
trigger(target, PAN_EVENT + DIRECTIONS[direct], event, detail)
//触发 panmove 事件
trigger(target, PAN_MOVE_EVENT, event, detail)
moveFlag = true
})
on(element, END_EVENT, function(event) {
// 取消 longtap 事件定时器
clearTime(longTapTimer)
var coords = getCoords(event), thresholdAndInterval
p2 = coords
p2.t = new Date()
thresholdAndInterval = getThresholdAndInterval(p1,p2)
// 如果达到 swipe 事件条件
if (matchSwipe(thresholdAndInterval.threshold, thresholdAndInterval.interval)) {
//触发 swipe['up', 'right', 'down', 'left'] 事件
trigger(target, SWIPE_EVENT + DIRECTIONS[findMatchedDirection(actions)], event)
} else if (!cancelTap && isTouch && matchTap(thresholdAndInterval.threshold, thresholdAndInterval.interval)) {
// 触发 tap 事件
trigger(target, TAP_EVENT, event)
}
// 触发 panend 事件
target && moveFlag && trigger(target, PAN_END_EVENT, event, getEventDetail(coords))
target = null
moveFlag = false
})
}
module.exports = Touch
<file_sep>/**
* # Kub
*
* `Kub` 对象。kub 框架对外暴露的全局变量,所有组件均绑在`Kub`对象中。
*
*/
var _window = window,
Kub = _window.Kub = _window.Kub || {}
Kub.version = '2.3.0'
/**
* ## Kub.$
*
* 类 `Zepto` 模块。
*/
Kub.$ = require('./lite')
/**
* ## Kub.core
*/
Kub.core = require('./core')
/**
* ## Kub.os
*/
Kub.os = require('./detect')
/**
* ## Kub.dateHelper
*/
Kub.dateHelper = require('./date')
/**
* ## Kub.cookie
*/
Kub.cookie = require('./cookie')
/**
* ## Kub.LazyLoad
*/
Kub.LazyLoad = require('./lazyload')
/**
* ## Kub.Dialog
*/
Kub.Dialog = require('./dialog')
/**
* ## Kub.Alert
*/
Kub.Alert = require('./alert')
/**
* ## Kub.Confirm
*/
Kub.Confirm = require('./confirm')
/**
* ## Kub.Prompt
*/
Kub.Prompt = require('./prompt')
/**
* ## Kub.Toast
*/
Kub.Toast = require('./toast')
/**
* ## Kub.Loader
*/
Kub.Loader = require('./loader')
/**
* ## Kub.Swiper
*/
Kub.Swiper = require('./swiper')
/**
* ## Kub.DatePicker
*/
Kub.DatePicker = require('./datepicker')
/**
* ## Kub.Touch
*/
Kub.Touch = require('./touch')
/**
* ## Kub.Popup
*/
Kub.Popup = require('./popup')
module.exports = Kub
<file_sep>/*! Kub Mobile JavaScript Components Library v2.4.0.*/
/******/ (function(modules) { // webpackBootstrap
/******/ // The module cache
/******/ var installedModules = {};
/******/ // The require function
/******/ function __webpack_require__(moduleId) {
/******/ // Check if module is in cache
/******/ if(installedModules[moduleId])
/******/ return installedModules[moduleId].exports;
/******/ // Create a new module (and put it into the cache)
/******/ var module = installedModules[moduleId] = {
/******/ exports: {},
/******/ id: moduleId,
/******/ loaded: false
/******/ };
/******/ // Execute the module function
/******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__);
/******/ // Flag the module as loaded
/******/ module.loaded = true;
/******/ // Return the exports of the module
/******/ return module.exports;
/******/ }
/******/ // expose the modules object (__webpack_modules__)
/******/ __webpack_require__.m = modules;
/******/ // expose the module cache
/******/ __webpack_require__.c = installedModules;
/******/ // __webpack_public_path__
/******/ __webpack_require__.p = "";
/******/ // Load entry module and return exports
/******/ return __webpack_require__(0);
/******/ })
/************************************************************************/
/******/ ([
/* 0 */
/***/ (function(module, exports, __webpack_require__) {
module.exports = __webpack_require__(6);
/***/ }),
/* 1 */,
/* 2 */,
/* 3 */,
/* 4 */,
/* 5 */,
/* 6 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # Kub
*
* `Kub` 对象。kub 框架对外暴露的全局变量,所有组件均绑在`Kub`对象中。
*
*/
var _window = window,
Kub = _window.Kub = _window.Kub || {}
Kub.version = '2.3.0'
/**
* ## Kub.$
*
* 类 `Zepto` 模块。
*/
Kub.$ = __webpack_require__(7)
/**
* ## Kub.core
*/
Kub.core = __webpack_require__(9)
/**
* ## Kub.os
*/
Kub.os = __webpack_require__(10)
/**
* ## Kub.dateHelper
*/
Kub.dateHelper = __webpack_require__(11)
/**
* ## Kub.cookie
*/
Kub.cookie = __webpack_require__(12)
/**
* ## Kub.LazyLoad
*/
Kub.LazyLoad = __webpack_require__(13)
/**
* ## Kub.Dialog
*/
Kub.Dialog = __webpack_require__(14)
/**
* ## Kub.Alert
*/
Kub.Alert = __webpack_require__(16)
/**
* ## Kub.Confirm
*/
Kub.Confirm = __webpack_require__(17)
/**
* ## Kub.Prompt
*/
Kub.Prompt = __webpack_require__(18)
/**
* ## Kub.Toast
*/
Kub.Toast = __webpack_require__(20)
/**
* ## Kub.Loader
*/
Kub.Loader = __webpack_require__(21)
/**
* ## Kub.Swiper
*/
Kub.Swiper = __webpack_require__(22)
/**
* ## Kub.DatePicker
*/
Kub.DatePicker = __webpack_require__(23)
/**
* ## Kub.Touch
*/
Kub.Touch = __webpack_require__(27)
/**
* ## Kub.Popup
*/
Kub.Popup = __webpack_require__(24)
module.exports = Kub
/***/ }),
/* 7 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # Lite
*
* 类似于`Zepto`,提供部分与Dom相关的方法,方法使用保持与`Zepto`一致。
*
*/
/**
* @require [polyfill](./polyfill.js.html)
*/
__webpack_require__(8)
var $, Lite
var ELEMENT_NODE = 1
var slice = Array.prototype.slice,
readyRE = /complete|loaded|interactive/,
idSelectorRE = /^#([\w-]+)$/,
classSelectorRE = /^\.([\w-]+)$/,
tagSelectorRE = /^[\w-]+$/,
spaceRE = /\s+/g
var _document = document,
_window = window,
_undefined = undefined
function wrap(dom, selector) {
dom = dom || []
Object.setPrototypeOf ? Object.setPrototypeOf(dom, $.fn) : (dom.__proto__ = $.fn)
dom.selector = selector || ''
return dom
}
var isArray = Array.isArray ||
function(object) {
return object instanceof Array
}
$ = Lite = function(selector, context) {
context = context || _document
var type = typeof selector
if (!selector) {
return wrap()
}
if (type === 'function') {
return $.ready(selector)
}
if (selector._l) {
return selector
}
if (isArray(selector)) {
//$([document,document.body])
return wrap(slice.call(selector).filter(function(item) {
return item != null
}), selector)
}
if (type === 'object') {
//$(document)
return wrap([selector], '')
}
if (type === 'string') {
selector = selector.trim()
if (selector[0] === '<') {
var nodes = $.fragment(selector)
return wrap(nodes, '')
}
if (idSelectorRE.test(selector)) {
var found = _document.getElementById(RegExp.$1)
return wrap(found ? [found] : [], selector)
}
return wrap($.qsa(selector, context), selector)
}
return wrap()
}
var createDelegator = function(handler, selector, element) {
return function(e) {
var match = $(e.target).closest(selector)
// 1、存在代理节点
// 2、排除$('.className').on('click','.className',handler) 情况
if (match.length && match[0] !== element) {
handler.apply(match[0], arguments)
}
}
}
!(function() {
this.qsa = function(selector, context) {
//querySelectorAll,如果存在两个相同ID,在ios7下,限定范围内查询 id 会返回两个节点
context = context || _document
selector = selector.trim()
return slice.call(classSelectorRE.test(selector) ? context.getElementsByClassName(RegExp.$1) : tagSelectorRE.test(selector) ? context.getElementsByTagName(selector) : context.querySelectorAll(selector))
}
this.fragment = function(html) {
var div = _document.createElement('div'),
nodes
div.innerHTML = html
nodes = div.children
div = null
return slice.call(nodes)
}
this.ready = function(callback) {
if (readyRE.test(_document.readyState) && _document.body) {
callback($)
} else {
_document.addEventListener('DOMContentLoaded', function() {
callback($)
}, false)
}
return this
}
this.Event = function(type, props) {
return new _window.CustomEvent(type, props)
}
this.fn = this.prototype = {
_l: true,
/**
* ## Lite.prototype.each
*
* 循环所有节点。
*/
each: function(callback) {
var l = this.length,
i, t
for (i = 0; i < l; i++) {
t = this[i]
if (callback.call(t, i, t) === false) {
return this
}
}
return this
},
/**
* ## Lite.prototype.slice
*
* 切割节点。
*/
slice: function() {
return $(slice.apply(this, arguments))
},
/**
* ## Lite.prototype.is
*
* 判断是否是指定的节点。
*/
is: function(selector, element) {
element = element ? element : this[0]
if (element && element.nodeType === ELEMENT_NODE) {
return element === selector ? true : typeof selector === 'string' && element.matches(selector)
}
return false
},
/**
* ## Lite.prototype.closest
*
* 查找最近的节点。
*
* 原生closest不包含本身,`jQuery`与`Zepto`包含本身,保持与`Zepto`一致。
*
*/
closest: function(selector) {
var element = this[0],dom
dom = element && typeof selector === 'string' ? element.closest(selector) : this.is(selector, element) ? element : null
return $(dom)
},
/**
* ## Lite.prototype.find
*
* 查找节点,只支持选择器查找.
*/
find: function(selector) {
var dom = []
this.each(function() {
if (this.nodeType !== ELEMENT_NODE) return
var elements = $.qsa(selector, this),
elementsLen = elements.length
for (var i = 0; i < elementsLen; i++) {
dom.indexOf(elements[i]) === -1 && dom.push(elements[i])
}
})
return $(dom)
},
/**
* ## Lite.prototype.show
*
* 显示。
*/
show: function() {
return this.each(function() {
this.style.display === 'none' && (this.style.display = '')
$(this).css('display') === 'none' && (this.style.display = 'block')
})
},
/**
* ## Lite.prototype.hide
*
* 隐藏。
*/
hide: function() {
return this.each(function() {
this.style.display = 'none'
})
},
/**
* ## Lite.prototype.css
*
* 修改或获取节点样式。
*/
css: function(property, value) {
var isObject = typeof property === 'object'
//get
if (value == null && !isObject) {
var el = this[0]
//not element
if (el.nodeType !== ELEMENT_NODE) return
return _window.getComputedStyle(el).getPropertyValue(property)
}
var css = ''
if (isObject) {
for (var key in property) {
property[key] == null ? this.each(function() {
this.style.removeProperty(key)
}) : (css += key + ':' + property[key] + ';')
}
} else {
css += key + ':' + property[key] + ';'
}
//set
css && this.each(function() {
this.style.cssText += ';' + css
})
return this;
},
/**
* ## Lite.prototype.offset
*
* 获取节点的`offset`,只支持获取,不支持设置。
*/
offset: function() {
if (!this.length) return null
var ele = this[0],
obj = ele.getBoundingClientRect()
//why window.pageXOffset
//http://www.cnblogs.com/hutaoer/archive/2013/02/25/3078872.html
return {
left: obj.left + _window.pageXOffset,
top: obj.top + _window.pageYOffset,
width: ele.offsetWidth,
height: ele.offsetHeight
}
},
/**
* ## Lite.prototype.addClass
*
* 添加`class`。
*/
addClass: function(name) {
if (name == _undefined) return this
return this.each(function() {
if (!('className' in this)) return
var classList = [],
className = this.className.trim(),
classNameList = className.split(spaceRE)
name.trim().split(spaceRE).forEach(function(klass) {
classNameList.indexOf(klass) === -1 && classList.push(klass)
})
classList.length && (this.className = (className && (className + ' ')) + classList.join(' '))
})
},
/**
* ## Lite.prototype.removeClass
*
* 移除`class`。
*/
removeClass: function(name) {
return this.each(function() {
if (!('className' in this)) return
if (name === _undefined) return this.className = ''
var className = this.className
name.trim().split(spaceRE).forEach(function(klass) {
//zepto
className = className.replace(new RegExp('(^|\\s)' + klass + '(\\s|$)', 'g'), ' ')
})
this.className = className
})
},
/**
* ## Lite.prototype.eq
*
* 获取指定索引节点。
*/
eq: function(idx) {
idx = idx < 0 ? idx + this.length : idx
return $(this[idx])
},
/**
* ## Lite.prototype.off
*
* 取消绑定事件,不支持移除代理事件。
*/
off: function(type, handler) {
var types = type && type.trim().split(spaceRE)
types && this.each(function() {
var element = this
types.forEach(function(name) {
if (handler) {
element.removeEventListener(name, handler.delegator || handler, false)
} else {
var handlers = element.listeners && element.listeners[name]
handlers && handlers.forEach(function(_handler) {
element.removeEventListener(name, _handler.delegator || _handler, false)
})
}
})
})
return this
},
/**
* ## Lite.prototype.on
*
* 监听事件,支持事件代理。
*
*/
on: function(type, selector, handler) {
var f = true
if (typeof selector !== 'string') {
f = false
handler = selector
}
if (typeof handler == 'function') {
var types = type && type.trim().split(spaceRE)
types && this.each(function() {
var element = this, listeners
if (f) {
handler.delegator = createDelegator(handler, selector, element)
}
listeners = element.listeners || {}
types.forEach(function(event) {
if (!listeners[event]) {
listeners[event] = []
}
listeners[event].push(handler)
element.addEventListener(event, handler.delegator || handler, false)
})
element.listeners = listeners
})
}
return this
},
/**
* ## Lite.prototype.trigger
*
* 触发事件。
*/
trigger: function(type, detail) {
return this.each(function() {
this && this.dispatchEvent && this.dispatchEvent($.Event(type, {
detail: detail,
bubbles: true,
cancelable: true
}))
})
},
/**
* ## Lite.prototype.attr
*
* 获取或者设置节点属性。
*/
attr: function(name, value) {
var result,
type = typeof name
if(type === 'string' && value == null) {
if(!this.length || this[0].nodeType !== ELEMENT_NODE){
return _undefined
}else{
return (!(result = this[0].getAttribute(name)) && name in this[0]) ? this[0][name] : result
}
}else{
return this.each(function() {
if (this.nodeType !== ELEMENT_NODE) return
if (type === 'object') {
for (key in name) {
this.setAttribute(key, name[key])
}
} else {
this.setAttribute(name, value)
}
})
}
},
/**
* ## Lite.prototype.removeAttr
*
* 移除节点属性。
*/
removeAttr: function(name) {
return this.each(function() {
var self = this
this.nodeType === ELEMENT_NODE && name.split(spaceRE).forEach(function(attribute) {
self.removeAttribute(attribute)
})
})
},
/**
* ## Lite.prototype.remove
*
* 删除节点。
*/
remove: function() {
return this.each(function() {
var parentNode = this.parentNode
parentNode && parentNode.removeChild(this)
})
},
/**
* ## Lite.prototype.appendTo
*
* 将html插入到Dom节点内底部。
*/
appendTo: function(target) {
var dom = [],
self = this
target.each(function() {
var node = this
self.each(function() {
dom.push(node.appendChild(this))
})
})
return $(dom)
},
/**
* ## Lite.prototype.after
*
* 在Dom节点之后插入html。
*/
after: function(html) {
return this.each(function() {
this.insertAdjacentHTML('afterend', html)
})
},
/**
* ## Lite.prototype.before
*
* 在Dom节点之前插入html。
*/
before: function(html) {
return this.each(function() {
this.insertAdjacentHTML('beforebegin', html)
})
},
/**
* ## Lite.prototype.append
*
* 在Dom节点内底部插入html。
*/
append: function(html) {
return this.each(function() {
this.insertAdjacentHTML('beforeend', html)
})
},
/**
* ## Lite.prototype.prepend
*
* 在Dom节点内头部插入html。
*/
prepend: function(html) {
return this.each(function() {
this.insertAdjacentHTML('afterbegin', html)
})
},
/**
* ## Lite.prototype.html
*
* 设置或获取Dom html。
*
*/
html: function(html) {
return html != _undefined ?
this.each(function() {
this.innerHTML = html
}) :
(this[0] ? this[0].innerHTML : null)
},
/**
* ## Lite.prototype.text
* 设置或获取Dom文本内容。
*/
text: function(text) {
return text != _undefined ?
this.each(function() {
this.textContent = text
}) :
(this[0] ? this[0].textContent : null)
}
}
}).call(Lite)
module.exports = _window.Zepto || Lite
/***/ }),
/* 8 */
/***/ (function(module, exports) {
var _window = window,
_document = document
var ELEMENT_NODE = 1,
ELEMENT_PROTOTYPE = Element.prototype
//CustomEvent polyfill
//android 4.3
if (!_window.CustomEvent) {
var CustomEvent = function(event, params) {
var evt
params = params || {
bubbles: false,
cancelable: false,
detail: undefined
}
evt = _document.createEvent("CustomEvent")
evt.initCustomEvent(event, params.bubbles, params.cancelable, params.detail)
return evt
}
CustomEvent.prototype = _window.Event.prototype
_window.CustomEvent = CustomEvent
}
//Element.prototype.matches polyfill
if (typeof ELEMENT_PROTOTYPE.matches !== 'function') {
ELEMENT_PROTOTYPE.matches = ELEMENT_PROTOTYPE.msMatchesSelector || ELEMENT_PROTOTYPE.mozMatchesSelector || ELEMENT_PROTOTYPE.webkitMatchesSelector || function matches(selector) {
var element = this,
// 查找当前节点的根节点时,必须使用element.ownerDocument
// 当节点插入到iframe中时,该节点的document就不是父窗口中的document,而是iframe中的document
// 会造成 document.querySelectorAll(selector) 查询不到改节点,所以需要element.ownerDocument替代document
elements = element.ownerDocument.querySelectorAll(selector),
index = 0
while (elements[index] && elements[index] !== element) {
++index
}
return !!elements[index]
}
}
//Element.prototype.closest polyfill
if (typeof ELEMENT_PROTOTYPE.closest !== 'function') {
ELEMENT_PROTOTYPE.closest = function closest(selector) {
var element = this
while (element && element.nodeType === ELEMENT_NODE) {
if (element.matches(selector)) {
return element
}
element = element.parentNode
}
return null
}
}
/***/ }),
/* 9 */
/***/ (function(module, exports) {
/**
* # core
*
* 核心模块,该模块提供基础方法。
*
*/
/**
* ## Core Constructor
*
* `Core` 模块,对外提供的是实例化的对象。
*
* 使用方法:
*
* ```js
*
* //获取url参数
* var params = Kub.core.getQuerystring()
*
* ```
*/
function Core() {
}
//解析 param string 正则表达式
var paramsRegxp = /([^=&]+)(=([^&#]*))?/g
var toString = Object.prototype.toString,
_window = window,
_href = _window.location.href,
_prototype = Core.prototype
//获取 params string
//url地址,未传值取 `window.location.href`。
var getParamsString = function(url) {
var matchs
url = url || _href
return url && (matchs = url.match(/^[^\?#]*\?([^#]*)/)) && matchs[1]
}
/**
* ## Core.prototype.extend
*
* @param {Boolean} [deep] `可选` 是否深度拷贝。
* @param {Object/Array} target 目标。
* @param {Object/Array} source 源对象,可为多个。
* @return {Object/Array} target
*/
_prototype.extend = function(target, source) {
var deep,
args = Array.prototype.slice.call(arguments, 1),
length
if (this.isBoolean(target)) {
deep = target
target = args.shift()
}
length = args.length
for (var i = 0; i < length; i++) {
source = args[i]
for (var key in source) {
if (source.hasOwnProperty(key)) {
if (deep && (this.isArray(source[key]) || this.isObject(source[key]))) {
if (this.isArray(source[key]) && !this.isArray(target[key])) {
target[key] = []
}
if (this.isObject(source[key]) && !this.isObject(target[key])) {
target[key] = {}
}
this.extend(deep, target[key], source[key])
} else {
(source[key] !== undefined) && (target[key] = source[key])
}
}
}
}
return target
}
/**
* ## Core.prototype.is[type]
*
* 类型检测函数。
*
* 具体类型`type`包含
*
* `['Arguments', 'Function', 'String', 'Number', 'Date', 'RegExp', 'Error', 'Array', 'Object', 'Boolean']`
*
*/
;
['Arguments', 'Function', 'String', 'Number', 'Date', 'RegExp', 'Error', 'Array', 'Object', 'Boolean'].forEach(function(name) {
_prototype['is' + name] = function(obj) {
return toString.call(obj) === '[object ' + name + ']'
}
})
/**
* ## Core.prototype.setQuerystring
*
* 设置 url 参数,如果 url 未传值,则默认取 `window.location.href`。
*
* 使用:
* ```js
*
* //设置当前地址参数
*
* //默认采用`window.location.href`
* Kub.core.setQuerystring({
* name:'kub'
* })
*
* //传入url
* Kub.core.setQuerystring('http://www.a.com?userId=123',{
* name:'kub'
* })
*
* //追加参数
*
* //如果不存在名称为 name 的参数,则新增参数。如果存在则替换其值
* Kub.core.setQuerystring({
* name:'kub',
* addr:'hangzhou'
* })
*
* ```
*
* @param {String} url url
*
* @param {Object} params 参数对象。
*
* @param {Object} opts 配置参数。
*
* - raw :配置是否开启 `encodeURIComponent`,默认为`false`,开启。
* - append :是否追加参数,默认为`true`。 true:如果 url 不存在当前参数名称,则追加一个参数。false:不追加,只进行替换。
*/
_prototype.setQuerystring = function(url, params, opts) {
//验证url是否传值,如果 url 未传值,则使用当前页面 url
if (this.isObject(url)) {
opts = params
params = url
url = _href
}
params = params || {}
opts = this.extend({
append: true,
raw: false
}, opts || {})
var queryString = getParamsString(url),
_queryString = '',
f = -1,
_params = {}
//解析 url 中的参数,存放在对象中
queryString && queryString.replace(paramsRegxp, function(a, name, c, value) {
if (params.hasOwnProperty(name)) {
value = params[name]
}
// 获取到的中文参数为编码后的,需decodeURIComponent解码
_params[name] = value != undefined ? (opts.raw ? value : decodeURIComponent(value)) : ''
})
//如果是追加,则合并参数
if (opts.append) {
for (var name in params) {
if (params.hasOwnProperty(name)) {
_params[name] = params[name] != undefined ? params[name] : ''
}
}
}
//将参数合并成字符串
for (name in _params) {
if (_params.hasOwnProperty(name)) {
_queryString += (++f ? '&' : '') + name + '=' + (_params[name] !== '' ? (opts.raw ? _params[name] : encodeURIComponent(_params[name])) : '')
}
}
//替换掉原来 url 中的 querystring
return url.replace(/^([^#\?]*)[^#]*/, function(a, url) {
return url + (_queryString ? '?' + _queryString : '')
})
}
/**
* ## Core.prototype.getQuerystring
*
* 获取url中的参数。
*
* 设置 url 参数,如果 url 未传值,则默认取 `window.location.href`。
*
* 使用:
* ```js
*
* //设置当前地址参数
*
* //默认采用`window.location.href`
* var params = Kub.core.getQuerystring()
*
* var name = params.name
*
* //传入url
* var params = Kub.core.getQuerystring('http://www.a.com?userId=123')
*
* var userId = params.userId
*
* ```
*
* @param {String} url url地址,未传值取 `window.location.href`。
*
* @param {Object} opts 配置参数。
*
* - raw :配置是否 `decodeURIComponent`,默认为`true`,开启。
*
* @return {Object} 返回参数对象
*/
_prototype.getQuerystring = function(url, opts) {
var href = _href
if (this.isObject(url)) {
opts = url
url = href
}
opts = this.extend({
raw: false
}, opts || {})
url = url || href
var params = {},
queryString = getParamsString(url)
queryString && queryString.replace(paramsRegxp, function(a, name, c, value) {
params[name] = opts.raw ? value : !!value ? decodeURIComponent(value) : undefined
})
return params
}
module.exports = new Core()
/***/ }),
/* 10 */
/***/ (function(module, exports) {
/**
* # os
*
* 检测系统类型与版本,包含系统类型与版本信息。
*
* 只检测Android 与 IOS, window phone(window phone 未进行完全测试)
*
* `os` 返回以下相关属性:
*
* `android` :是否是Android
*
* `ios` :是否是IOS系统
*
* `ipad` :是否是iPad
*
* `iphone` :是否是iPhone
*
* `ipod` :是否是iPod
*
* `mobile` :是否是移动端
*
* `phone` :是否是手机
*
* `tablet` :是否是平板
*
* `version` :系统版本
*
* `wp` : 是否是window phone
*
*/
var ua = navigator.userAgent,
android = ua.match(/(Android);?[\s\/]+([\d.]+)?/),
ipad = ua.match(/(iPad).*OS\s([\d_]+)/),
ipod = ua.match(/(iPod)(.*OS\s([\d_]+))?/),
iphone = !ipad && ua.match(/(iPhone\sOS)\s([\d_]+)/),
wp = ua.match(/Windows Phone ([\d.]+)/),
os = {}
//android
android ? (os.android = true, os.version = android[2]) : (os.android = false)
//iphone
iphone && !ipod ? ( os.ios = os.iphone = true, os.version = iphone[2].replace(/_/g, '.') ) : (os.iphone = false)
//ipad
ipad ? ( os.ios = os.ipad = true, os.version = ipad[2].replace(/_/g, '.') ) : (os.ipad = false)
//ipod
ipod ? ( os.ios = os.ipod = true, os.version = ipod[3].replace(/_/g, '.') ) : (os.ipod = false)
//window phone
wp ? ( os.wp = true, os.version = wp[1]) : (os.wp = false)
//ios
!os.iphone && !os.ipad && !os.ipod && (os.ios = false)
//手机
os.phone = os.android && /mobile/i.test(ua) || os.iphone || os.wp ? true : false
//平板
os.tablet = !os.phone && ( os.android || os.ipad || /window/i.test(ua) && /touch/i.test(ua) ) ? true : false
//移动端
os.mobile = os.phone || os.tablet
module.exports = os
/***/ }),
/* 11 */
/***/ (function(module, exports) {
/**
* # DateHelper
*
* 日期格式化组件。
*
* 格式化字符:
*
yyyy : 四位年。例如:2015
yy : 二位年份,最后两位。例如 15
MMMM : 全称月份。例如 January
MMM : 简称月份。例如 Jan
MM : 两位月份,小于10显示03。例如:11
M : 一位月份,小于10显示3.例如:3或者11
dddd : 全称星期。例如:星期一,Sunday
ddd : 简称星期。例如:一,Sun
dd : 两位天数。类似于MM
d : 一位天数。类似于M
HH : 两位小时。类似于MM
H : 一位小时。类似于M
mm : 两位分钟。类似于MM
m : 一位分钟。类似于M
ss : 两位秒数。类似于MM
s : 一位秒数。类似于M
aa : 全称上午或者下午。例如A.M.,P.M.
a : 简称上午或者下午。例如AM.
*/
/**
* ## DateHelper Constructor
*
* `DateHelper` 模块,对外提供的是实例化的对象。
*
* 使用:
* ```js
* //String to Date
*
* '2015-05-20'.parseDate('yyyy-MM-dd')
*
* //格式化日期
* new Date().format('yyyy-MM-dd,HH:mm:ss')
*
* ```
*/
function DateHelper() {
}
var _prototype = DateHelper.prototype
var get2Year = function(date) {
return (date.getFullYear() + '').replace(/\d{2}$/, '00') - 0
}
var get2 = function(value) {
return value < 10 ? '0' + value : value
}
var getAmPm = function(date) {
return date.getHours() < 12 ? 0 : 1
}
//获取相对应的日期相关数据
var getValueByPattern = function(datehelper, fmt, date) {
var patterns = {
yyyy: date.getFullYear(),
yy: date.getFullYear() - get2Year(date),
MMMM: datehelper.i18n[datehelper.locale].month.full[date.getMonth()],
MMM: datehelper.i18n[datehelper.locale].month.abbr[date.getMonth()],
MM: get2(date.getMonth() + 1),
M: date.getMonth() + 1,
dddd: datehelper.i18n[datehelper.locale].day.full[date.getDay()],
ddd: datehelper.i18n[datehelper.locale].day.abbr[date.getDay()],
dd: get2(date.getDate()),
d: date.getDate(),
HH: get2(date.getHours()),
H: date.getHours(),
mm: get2(date.getMinutes()),
m: date.getMinutes(),
ss: get2(date.getSeconds()),
s: date.getSeconds(),
aa: datehelper.i18n[datehelper.locale].amPm.full[getAmPm(date)],
a: datehelper.i18n[datehelper.locale].amPm.abbr[getAmPm(date)]
}
return patterns[fmt]
}
//本地化,目前包含`en`与`zh`
_prototype.i18n = {
en: {
month: {
abbr: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'],
full: ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December']
},
day: {
abbr: ['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat'],
full: ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']
},
amPm: {
abbr: ['AM', 'PM'],
full: ['A.M.', 'P.M.']
}
},
zh: {
month: {
abbr: ['一月', '二月', '三月', '四月', '五月', '六月', '七月', '八月', '九月', '十月', '十一月', '十二月'],
full: ['一月份', '二月份', '三月份', '四月份', '五月份', '六月份', '七月份', '八月份', '九月份', '十月份', '十一月份', '十二月份']
},
day: {
abbr: ['日', '一', '二', '三', '四', '五', '六'],
full: ['星期日', '星期一', '星期二', '星期三', '星期四', '星期五', '星期六']
},
amPm: {
abbr: ['上午', '下午'],
full: ['上午', '下午']
}
}
}
//默认中文
_prototype.locale = 'zh'
/**
* ## DateHelper.prototype.addLocale
*
* 添加本地化
*
* @param {String} name 本地化名称
* @param {Object} locale 本地化数据
* @return {instance} 当前实例
*/
_prototype.addLocale = function(name, locale) {
name && locale && (this.i18n[name] = locale)
return this
}
/**
* ## DateHelper.prototype.setLocale
*
* 设置默认本地化
*
* @param {String} name 本地化名称
* @return {instance} 当前实例
*/
_prototype.setLocale = function(name) {
this.locale = name
return this
}
/**
* ## DateHelper.prototype.format
*
* 格式化日期。
*
* @param {Date} date 日期
* @param {String} format 日期格式
* @return {String} 格式化以后的日期
*/
_prototype.format = function(date, format) {
var self = this
if (!date) return
format = format || 'yyyy-MM-dd'
format = format.replace(/(yyyy|yy|MMMM|MMM|MM|M|dddd|ddd|dd|d|HH|H|mm|m|ss|s|aa|a)/g, function(part) {
return getValueByPattern(self, part, date)
})
return format
}
/**
* ## DateHelper.prototype.parse
*
* 转换日期。
*
* 此方法存在一个BUG,例如:
*
* ```js
*
* //1112会被计算在MM内。
* dateHelper.parse('2015-1112','yyyy-MMdd')
*
*
* //所以在使用parse方法时,每一个串使用字符分隔开。类似于:
*
* dateHelper.parse('2015-11-12','yyyy-MM-dd')
*
* ```
*
* @param {String} input 字符串
* @param {String} format 格式化字符串
* @return {Date} 格式化的日期
*/
_prototype.parse = function(input, format) {
if (!input || !format) return
var parts = input.match(/(\d+)/g),
i = 0,
fmt = {}
// extract date-part indexes from the format
format.replace(/(yyyy|yy|MM|M|dd|d|HH|H|mm|m|ss|s)/g, function(part) {
fmt[part] = i++
})
var year = parts[fmt['yyyy']] || (parseInt(parts[fmt['yy']], 10) + get2Year(new Date())) || 0,
month = (parts[fmt['MM']] - 1) || (parts[fmt['M']] - 1) || 0,
day = parts[fmt['dd']] || parts[fmt['d']] || 0,
hour = parts[fmt['HH']] || parts[fmt['H']] || 0,
minute = parts[fmt['mm']] || parts[fmt['m']] || 0,
second = parts[fmt['ss']] || parts[fmt['s']] || 0
return new Date(year, month, day, hour, minute, second)
}
var dateHelper = new DateHelper()
// 将 parseDate 方法绑定在 `String` 原型上
String.prototype.parseDate = function(format) {
return dateHelper.parse(this, format)
}
// 将 format 方法绑定在 `Date` 原型上
Date.prototype.format = function(format) {
return dateHelper.format(this, format)
}
module.exports = dateHelper
/***/ }),
/* 12 */
/***/ (function(module, exports) {
/**
* # cookie
*
* 操作cookie方法,`expires` 如果为数字,单位为`毫秒`。
*
* 使用方法:
* ```js
* //取值
* var name = Kub.cookie('name')
*
* //设置值
* Kub.cookie('name','kub')
*
* //配置cookie相关属性
* Kub.cookie('name','kub',{
* domain:'.a.com'
*
* })
* ```
*
*/
var _document = document,
_encodeURIComponent = encodeURIComponent
function cookie(key, value, options) {
var days, time, result, decode
options = options || {}
if(!options.hasOwnProperty('path')){
options.path = '/'
}
// A key and value were given. Set cookie.
if (arguments.length > 1 && String(value) !== "[object Object]") {
// Enforce object
if (value === null || value === undefined) options.expires = -1
if (typeof options.expires === 'number') {
days = options.expires
time = options.expires = new Date()
time.setTime(time.getTime() + days)
}
value = String(value)
return (_document.cookie = [
_encodeURIComponent(key), '=',
options.raw ? value : _encodeURIComponent(value),
options.expires ? '; expires=' + options.expires.toUTCString() : '',
options.path ? '; path=' + options.path : '',
options.domain ? '; domain=' + options.domain : '',
options.secure ? '; secure' : ''
].join(''))
}
// Key and possibly options given, get cookie
options = value || {}
decode = options.raw ? function (s) { return s } : decodeURIComponent
return (result = new RegExp('(?:^|; )' + _encodeURIComponent(key) + '=([^;]*)').exec(_document.cookie)) ? decode(result[1]) : null
}
module.exports = cookie
/***/ }),
/* 13 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # LazyLoad
*
* 延迟加载组件。
*
*/
/**
* @require [core](./core.js.html)
* @require [Lite](./lite.js.html)
*/
var core = __webpack_require__(9),
$ = __webpack_require__(7)
/**
* ## LazyLoad Constructor
*
* `LazyLoad` 构造函数。
*
* 使用:
* ```js
* var lazyload = new Kub.LazyLoad($('img'))
* ```
*/
function LazyLoad(element, options) {
this.$element = $(element)
this.options = core.extend({}, _prototype.defaults, options || {})
this.$container = $(this.options.container)
this.isWebp = this.isSupportWebp()
init(this)
}
var _window = window,
_prototype = LazyLoad.prototype
var //横竖屏切换时 ,orientationchange 与 resize事件都会触发,所以只需监听 resize 即可
RESIZE_EVENT = 'resize',
//三星某款机型在监听非window容器的scroll事件时,会无限次触发,可以使用touchmove替代
//但可能会出现 页面加载时触发scroll无法触发scroll与惯性滚动停止时,无法触发的情况
//综上:依旧采用scroll解决,对于某些机型进行忽略
EVENT_NAME = 'scroll'
//获取所有还未被加载的节点
var getUnloadedElements = function(lazyload) {
var dom = []
lazyload.$element.each(function() {
!this.loaded && dom.push(this)
})
return dom
}
//加载所有在可视区域内的图片
var loadElementsInViewport = function(lazyload) {
var elements
elements = getUnloadedElements(lazyload)
lazyload.completed = elements.length === 0 ? true : false
elements.forEach(function(element) {
var $this = $(element)
lazyload.isVisible($this) && lazyload.load($this)
})
}
// 图片格式转换成webp格式
var handleImgToWebp = function(url, lazyload) {
var changeURL = url.toString().replace(/(\.(?:gif|jpg|jpeg|png)(?:\.(?:jpg|png|webp))?)/i, function(match) {
var result = ''
if (match) {
var type = match.toLowerCase()
}
switch (type) {
case '.png.webp':
case '.jpg.webp':
case '.gif.webp':
case '.jpeg.webp':
result = match;
break;
case '.png':
case '.jpg':
case '.jpeg':
result = lazyload.isWebp ? match + '.webp' : match;
break;
case '.gif':
result = match
break;
}
return result
});
return changeURL
}
var init = function(lazyload) {
var options = lazyload.options,
timer
var handler = function() {
if (lazyload.completed) {
return
}
timer && clearTimeout(timer)
timer = setTimeout(function() {
loadElementsInViewport(lazyload)
}, options.delay)
}
//页面载入先执行下面
loadElementsInViewport(lazyload)
//页面紧接着触发scroll,走下面监听
lazyload.$container.on(EVENT_NAME, handler)
$(_window).on(RESIZE_EVENT, handler)
}
/**
* ## LazyLoad.prototype.defaults
*
* 默认配置项。
*
* 配置项说明:
*
* `container` : `Selector` 图片存放的容器,容器会监听事件。
*
* `threshold` : `Number` 提前加载距离。
*
* `delay` : `Number` 事件监听时的延迟时间。
*
* `attributeName` : `String` 属性名称。默认会从`element`上取出 `data-original` 属性。
*
* `load` : `Function` 图片加载事件。
*
* `useWebp` : `Boolean` 加载图片是否使用webp格式。默认为false:不使用webp格式。
*
*/
_prototype.defaults = {
container: _window,
threshold: 200,
delay: 100,
attributeName: 'original',
load: null,
useWebp: false
}
/**
* ## LazyLoad.prototype.updateElement
*
* 更新需要加载的节点,更新以后会立即检测是否有节点在可视区域内,修改的节点为替换,不是增加,意味着原来的节点会被替换。
*
* @return {instance} 当前实例
*/
_prototype.updateElement = function(element) {
this.$element = $(element)
//更新 dom 以后立即验证是否有元素已经显示
loadElementsInViewport(this)
return this
}
/**
* ## LazyLoad.prototype.loadAll
*
* 强制加载所有图片,无论节点是否在可视区域内。
*
* @return {instance} 当前实例
*/
_prototype.loadAll = function() {
var self = this,
elements
elements = getUnloadedElements(self)
this.completed = true
elements.forEach(function(element) {
var $this = $(element)
self.load($this)
})
return self
}
/**
* ## LazyLoad.prototype.isVisible
*
* 是否在视窗可见。
* @param {$} $this 元素
* @param {Object} options 参数
* @return {Boolean} true :可见 false :不可见
*/
_prototype.isVisible = function($this) {
var options = this.options,
element = $this[0]
//如果节点不可见,则不进行加载
if (element.offsetWidth == 0 && element.offsetHeight == 0 && element.getClientRects().length == 0) {
return false
}
if (this.abovethetop($this, options)) {
return false
} else if (this.belowthefold($this, options)) {
return false
}
if (this.leftofbegin($this, options)) {
return false
} else if (this.rightoffold($this, options)) {
return false
}
return true
}
/**
* ## LazyLoad.prototype.isSupportWebp
*
* 是否支持webp图片格式后缀。
*
* @return {Boolean} true: 支持 false: 不支持
*/
_prototype.isSupportWebp = function(){
var element = document.createElement('canvas')
if (!!(element.getContext && element.getContext('2d'))) {
return element.toDataURL('image/webp').indexOf('data:image/webp') == 0
} else {
return false
}
}
/**
* ## LazyLoad.prototype.load
*
* 加载指定元素。
*
* @param {$} $element 加载的节点
* @param {String} original 图片地址
* @return {instance} 当前实例
*/
_prototype.load = function($element) {
var options = this.options,
original = $element.attr('data-' + options.attributeName),
load = options.load
//如果原图片为空
if (!original) {
return this
}
original = options.useWebp ? handleImgToWebp(original, this) : original
if ($element[0].nodeName === 'IMG') {
$element.attr('src', original)
} else {
$element.css('background-image', 'url(' + original + ')')
}
//记录该节点已被加载
$element[0].loaded = true
// 触发 load 事件
load && load.call($element[0])
return this
}
/**
* ## LazyLoad.prototype.belowthefold
*
* 是否在视窗下面。
*
* @param {Element} element 检查的元素
* @param {Object} settings 被检查时的参数
* @return {Boolean} 是:true 否 :false
*/
_prototype.belowthefold = function(element, settings) {
var fold, container = settings.container
if (container === _window) {
fold = _window.innerHeight + _window.scrollY
} else {
var $container = $(container),
offset = $container.offset()
fold = offset.top + $container[0].offsetHeight
}
return fold <= $(element).offset().top - settings.threshold
}
/**
* ## LazyLoad.prototype.abovethetop
*
* 是否在视窗上面。
*
* @param {Element} element 检查的元素
* @param {Object} settings 被检查时的参数
* @return {Boolean} 是:true 否 :false
*/
_prototype.abovethetop = function(element, settings) {
var fold, container = settings.container
if (container === _window) {
fold = _window.scrollY
} else {
fold = $(container).offset().top
}
var $element = $(element),
offset = $element.offset()
return fold >= offset.top + settings.threshold + $element[0].offsetHeight
}
/**
* ## LazyLoad.prototype.rightoffold
*
* 是否在视窗右侧。
*
* @param {Element} element 检查的元素
* @param {Object} settings 被检查时的参数
* @return {Boolean} 是:true 否 :false
*/
_prototype.rightoffold = function(element, settings) {
var fold, container = settings.container
if (container === _window) {
fold = _window.innerWidth + _window.scrollX
} else {
var $container = $(container),
offset = $container.offset()
fold = offset.left + $container[0].offsetWidth
}
return fold <= $(element).offset().left - settings.threshold
}
/**
* ## LazyLoad.prototype.leftofbegin
*
* 是否在视窗左侧。
*
* @param {Element} element 检查的元素
* @param {Object} settings 被检查时的参数
* @return {Boolean} 是:true 否 :false
*/
_prototype.leftofbegin = function(element, settings) {
var fold, container = settings.container
if (container === _window) {
fold = _window.scrollX
} else {
fold = $(container).offset().left
}
var $element = $(element),
offset = $element.offset()
return fold >= offset.left + settings.threshold + $element[0].offsetWidth
}
module.exports = LazyLoad
/***/ }),
/* 14 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # Dialog
*
* 对话框.
*/
/**
* @require [core](./core.js.html)
* @require [Lite](./lite.js.html)
*/
var core = __webpack_require__(9),
$ = __webpack_require__(7),
template = __webpack_require__(15)
/**
* ## Dialog Constructor
*
* Dialog 构造函数。
*
* 使用:
* ```js
* //可定制多个按钮
* var dialog = new Kub.Dialog({
* message:'这是个弹窗',
* title:'弹窗',
* buttons:[{
* text:'确定',
* handler:function(e,dialog){
*
* }
* },{
* text:'取消',
* handler:function(e,dialog){
* //返回 event 与 dialog对象
* dialog.close()
* }
* }]
* })
* ```
*/
function Dialog(options) {
this.options = core.extend({}, _prototype.defaults, options || {})
init(this)
}
var ZOOMIN_CLASS = 'kub-animated kub-zoomin',
DIALOG_SELECTOR = '.J_dialog',
DIALOG_BUTTON_SELECTOR = '.J_dialogButton',
EVENT_NAME = 'click'
var $body
var _prototype = Dialog.prototype
var render = function(dialog,data) {
var html = template(data)
dialog.$element = $(html).appendTo($body)
return this
}
var bindEvents = function(dialog){
var options = dialog.options
//注册按钮事件
dialog.$element.find(DIALOG_BUTTON_SELECTOR).on(EVENT_NAME, function(e) {
var index = parseInt($(this).attr('data-index')),
button = options.buttons[index]
button.handler && button.handler.call(this, e, dialog)
})
}
var init = function(dialog) {
!$body && ($body = $(document.body))
//渲染数据
render(dialog, dialog.options)
dialog.$dialog = dialog.$element.find(DIALOG_SELECTOR)
dialog.setPosition && dialog.setPosition()
dialog.show()
bindEvents(dialog)
}
/**
* ## Dialog.prototype.defaults
*
* 默认配置项。
*
* 配置项说明:
*
* * `modal` : `Boolean` 是否显示遮罩层。
*
* * `title` : `String` 对话框名称。
*
* * `showHeader` : `Boolean` 是否显示头部。
*
* * `message` : `String` 弹窗内容。
*
* * `className` : `String` 弹窗类名。
*
* * `animated` : `Boolean` 是否开启动画效果。
*
* * `buttons`: `Array` 弹窗按钮。
*
* ```js
* //例如:
* [{
* text:'按钮名称',//按钮名称
* className:'button-name',//按钮class类名
* handler:function(){
* //按钮单击触发事件
* }
* }]
* ```
*/
_prototype.defaults = {
modal: true,
title: '',
showHeader: true,
message: '',
className: '',
animated: true,
buttons: null
}
/**
* ## Dialog.prototype.show
*
* 显示弹窗。
* @return {instance} 返回当前实例
*/
_prototype.show = function() {
this.$element.show()
this.options.animated && this.$dialog.addClass(ZOOMIN_CLASS)
return this
}
/**
* ## Dialog.prototype.hide
*
* 隐藏弹窗。
* @return {instance} 返回当前实例
*/
_prototype.hide = function() {
this.$element.hide()
this.options.animated && this.$dialog.removeClass(ZOOMIN_CLASS)
return this
}
/**
* ## Dialog.prototype.close
*
* 关闭弹窗。
* @return {instance} 返回当前实例
*/
_prototype.close = function() {
var opts = this.options
if (opts.closeHandler && opts.closeHandler.call(this) === false) {
return this
}
this.hide()
this.$element.remove()
return this
}
module.exports = Dialog
/***/ }),
/* 15 */
/***/ (function(module, exports) {
module.exports = function(data){
var __t,__p='',__j=Array.prototype.join,print=function(){__p+=__j.call(arguments,'');};
__p+='<div class="kub-dialog-modal '+
((__t=( data.className))==null?'':__t)+
' ';
if( data.modal ){
__p+=' kub-modal ';
}
__p+='"><div class="kub-dialog-wrapper"><div class="kub-dialog-container"><div class="kub-dialog J_dialog"> ';
if(data.showHeader){
__p+=' <div class="kub-dialog-header"> '+
((__t=( data.title))==null?'':__t)+
' </div> ';
}
__p+=' <div class="kub-dialog-body"> '+
((__t=( data.message))==null?'':__t)+
' </div> ';
if(data.buttons && data.buttons.length){
__p+=' <div class="kub-dialog-footer"> ';
for (var i=0,j=data.buttons.length;i<j;i++){
__p+=' <button class="kub-dialog-button J_dialogButton '+
((__t=( data.buttons[i].className || ''))==null?'':__t)+
'" data-index="'+
((__t=( i))==null?'':__t)+
'"> '+
((__t=( data.buttons[i].text))==null?'':__t)+
' </button> ';
}
__p+=' </div> ';
}
__p+=' </div></div></div></div>';
return __p;
};
/***/ }),
/* 16 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # Alert
*
* alert弹窗。
*/
/**
* @require [core](./core.js.html)
* @extend [Dialog](./dialog.js.html)
*/
var core = __webpack_require__(9),
Dialog = __webpack_require__(14)
/**
* ## Alert Constructor
*
* 继承于`Dialog`,可使用`Dialog`类中的方法。
*
* 使用方法:
* ```js
* var alert = new Kub.Alert()
* ```
*/
function Alert(options) {
var opts = this.options = core.extend({}, _prototype.defaults, options || {})
opts.buttons = [{
text: opts.confirmText,
handler: this.options.confirm || function(e, dialog) {
dialog.close()
}
}]
Dialog.call(this, opts)
}
var _prototype = Alert.prototype = Object.create(Dialog.prototype)
_prototype.constructor = Alert
/**
* ## Alert.defaults
*
* `Alert` 默认配置项。
*
* 配置项说明:
*
* * `confirmText` : `String` 确认按钮名称。
*
* * `confirm` : `Function` 单击确认按钮时触发的事件。
*
* > 如果未传递,单击时会默认关闭弹窗。
* >
* > 如果传递,需调用 `dialog.close()`关闭弹窗。
*
* * `showHeader` : `Boolean` 是否显示头部。
*
* * `className` : `String` 弹窗类名,修改时需加上`kub-alert`默认类名。
*
* * `modal` : `Boolean` 是否显示遮罩层。
*/
_prototype.defaults = {
confirmText: '确定',
confirm: null,
showHeader: false,
className: 'kub-alert',
modal: true
}
module.exports = Alert
/***/ }),
/* 17 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # Confirm
*
* confirm 弹窗。
*/
/**
* @require [core](./core.js.html)
* @extend [Dialog](./dialog.js.html)
*/
var core = __webpack_require__(9),
Dialog = __webpack_require__(14)
/**
* ## Confirm Constructor
*
* 继承于`Dialog`,可使用`Dialog`类中的方法。
*
* 使用方法:
* ```js
* var confirm = new Kub.Confirm({
* confirm:function(e,dialog){
* //do something
* dialog.close()
* }
* })
* ```
*/
function Confirm(options) {
var opts = this.options = core.extend({}, _prototype.defaults, options || {})
opts.buttons = [{
text: opts.cancelText,
handler: opts.cancel || function(e, dialog) {
dialog.close()
}
}, {
text: opts.confirmText,
handler: opts.confirm
}]
Dialog.call(this, opts)
}
var _prototype = Confirm.prototype = Object.create(Dialog.prototype)
_prototype.constructor = Confirm
/**
* ## Confirm.defaults
*
* `Confirm`默认配置项。
*
* 配置项说明:
*
* * `confirmText` : `String` 确认按钮名称。
*
* * `confirm` : `Function` 单击确认按钮时触发的事件。一般用于用户单击确认按钮执行事件。需调用`dialog.close()`手动关闭弹窗。
*
* * `cancelText` : `String` 取消按钮名称。
*
* * `cancel` : `Function` 单击取消按钮时触发的事件。
*
* > 如果未传递,单击时会默认关闭弹窗。
* >
* > 如果传递,需调用 `dialog.close()`关闭弹窗。
*
* * `showHeader` : `Boolean` 是否显示头部。
*
* * `className` : `String` 弹窗类名,修改时需加上`kub-confirm`默认类名。
*
* * `modal` : `Boolean` 是否显示遮罩层。
*/
_prototype.defaults = {
confirmText: '确定',
confirm: null,
cancelText: '取消',
cancel: null,
showHeader: false,
className: "kub-confirm",
modal: true
}
module.exports = Confirm
/***/ }),
/* 18 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # Prompt
*
* prompt 输入框。
*/
/**
* @require [core](./core.js.html)
* @extend [Dialog](./dialog.js.html)
*/
var core = __webpack_require__(9),
Dialog = __webpack_require__(14),
template = __webpack_require__(19)
var INPUT_SELECTOR = '.J_input'
/**
* ## Prompt Constructor
*
* 继承于`Dialog`,可使用`Dialog`类中的方法。
*
* 使用方法:
* ```js
* var prompt = new Kub.Prompt({
* confirm:function(event,dialog,value){
* //输入框输入的值 value
* }
* })
* ```
*/
function Prompt(options) {
var opts = this.options = core.extend({}, _prototype.defaults, options || {})
opts.buttons = [{
text: opts.cancelText,
handler: opts.cancel || function(e, dialog) {
dialog.close()
}
}, {
text: opts.confirmText,
handler: function(e, dialog) {
var value = dialog.$element.find(INPUT_SELECTOR)[0].value
opts.confirm && opts.confirm.call(this, e, dialog, value)
}
}]
opts.message = template(opts)
Dialog.call(this, opts)
}
var _prototype = Prompt.prototype = Object.create(Dialog.prototype)
_prototype.constructor = Prompt
/**
* ## Prompt.prototype.defaults
*
* `Prompt`默认配置项。
*
* 配置项说明:
*
* * `confirmText` : `String` 确认按钮名称。
*
* * `confirm` : `Function` 单击确认按钮时触发的事件。用于用户单击确认按钮执行事件。需调用`dialog.close()`手动关闭弹窗。
*
* * `cancelText` : `String` 取消按钮名称。
*
* * `cancel` : `Function` 单击取消按钮时触发的事件。
*
* > 如果未传递,单击时会默认关闭弹窗。
* >
* > 如果传递,需调用 `dialog.close()`关闭弹窗。
*
* * `showHeader` : `Boolean` 是否显示头部。
*
* * `className` : `String` 弹窗类名,修改时需加上`kub-prompt`默认类名。
*
* * `modal` : `Boolean` 是否显示遮罩层。
*
* * `message` : `String` 提示内容。
*
* * `inputType` : `String` 输入框类型。
*
* * `placeholder` : `String` 输入框 `placeholder` 属性。
*
* * `defaultValue` : `String` 输入框默认值。
*/
_prototype.defaults = {
confirmText: '确定',
confirm: null,
cancelText: '取消',
cancel: null,
showHeader: false,
className: 'kub-prompt',
modal: true,
message:'',
inputType: 'text',
placeholder: '',
defaultValue: ''
}
module.exports = Prompt
/***/ }),
/* 19 */
/***/ (function(module, exports) {
module.exports = function(data){
var __t,__p='',__j=Array.prototype.join,print=function(){__p+=__j.call(arguments,'');};
__p+=''+
((__t=(data.message))==null?'':__t)+
' <div class="kub-prompt-input-wrapper"><input placeholder="'+
((__t=( data.placeholder))==null?'':__t)+
'" type="'+
((__t=( data.inputType))==null?'':__t)+
'" value="'+
((__t=( data.defaultValue))==null?'':__t)+
'" class="kub-prompt-input J_input"></div>';
return __p;
};
/***/ }),
/* 20 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # Toast
*
* 提示框
*/
/**
* @require [core](./core.js.html)
* @extend [Dialog](./dialog.js.html)
*/
var core = __webpack_require__(9),
Dialog = __webpack_require__(14)
/**
* ## Toast Constructor
*
* 继承于`Dialog`,可使用`Dialog`类中的方法。
*
* 使用方法:
* ```js
* var toast = new Kub.Toast({
* message:'操作成功。'
* })
* ```
*/
function Toast(options){
var self = this,
opts = this.options = core.extend({}, _prototype.defaults, options||{},{
showHeader:false,
buttons:null
})
Dialog.call(this, opts)
//自动关闭
setTimeout(function(){
self.close()
}, opts.delay)
}
var _prototype = Toast.prototype = Object.create(Dialog.prototype)
_prototype.constructor = Toast
/**
* ## Toast.prototype.defaults
*
* `Toast`默认配置项。
*
* 配置项说明:
*
* * `message` : `String` 提示内容。
*
* * `className` : `String` 弹窗类名,修改时需加上`kub-toast`默认类名。
*
* * `top` : `Number` 距离顶部高度。
*
* * `delay` : `Number` 延迟时间。
*
* * `modal` : `Boolean` 是否显示遮罩层。
*/
_prototype.defaults = {
message:'',
className:'kub-toast',
top:50,
delay:2000,
modal:false
}
_prototype.setPosition = function(){
var top = this.options.top
this.$element.css({
top:core.isNumber(top) ? top + 'px' : top
})
return this
}
module.exports = Toast
/***/ }),
/* 21 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # Loader
*
* 加载等待框。
*
*/
/**
* @require [core](./core.js.html)
* @extend [Dialog](./dialog.js.html)
*/
var core = __webpack_require__(9),
Dialog = __webpack_require__(14)
/**
* ## Loader Constructor
*
* 继承于`Dialog`,可使用`Dialog`类中的方法。
*
* 使用方法:
*
* ```js
* var loader = new Kub.Loader()
*
* //隐藏loader
* loader.hide()
*
* var loader = new Kub.Loader({
* message: '定制提示内容'
* })
*
* ```
*/
function Loader(options) {
var opts = this.options = core.extend({}, _prototype.defaults, options || {}, {
showHeader: false,
buttons: null
})
Dialog.call(this, opts)
}
var _prototype = Loader.prototype = Object.create(Dialog.prototype)
_prototype.constructor = Loader
/**
* ## Loader.prototype.defaults
*
* `Loader` 默认配置项。
*
* 配置项说明:
*
* * `className`: `String` 弹窗类名,修改时需加上`kub-loader`默认类名。
*
* * `message` : `String` 提示内容。
*
* * `modal` : `Boolean` 是否显示遮罩层。
*/
_prototype.defaults = {
className: 'kub-loader',
modal: true,
message: '加载中…'
}
module.exports = Loader
/***/ }),
/* 22 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # Swiper
*
* 切换组件
*/
/**
* @require [core](./core.js.html)
* @require [os](./detect.js.html)
* @require [Lite](./lite.js.html)
*/
var core = __webpack_require__(9),
os = __webpack_require__(10),
$ = __webpack_require__(7)
/**
* ## Swiper Constructor
*
* `Swiper`类。
*
* 使用方法:
* ```js
* new Kub.Swiper('.swiper',{
* slideSelector:'.slide',
* slideActiveClass:'active',
* paginationSelector:'.pagination li',
* paginationActiveClass:'pagination-active',
* slide:function(index){
* //当前滚动索引
* }
* })
* ```
*/
function Swiper(element, options) {
this.options = core.extend(true, {}, _prototype.defaults, options || {})
this.$element = $(element)
var ui = this._ui = {
slides: $(options.slideSelector),
paginations: $(options.paginationSelector)
}
ui.slidesLength = ui.slides.length
init(this)
}
var $document = $(document),
isTouch = os.mobile
var START_EVENT = isTouch ? 'touchstart' : 'mousedown',
MOVE_EVENT = isTouch ? 'touchmove' : 'mousemove',
END_EVENT = isTouch ? 'touchend' : 'mouseup',
RESIZE_EVENT = 'resize',
TRANSITIONEND_EVENT = 'transitionend',
WEBKIT_TRANSITIONEND_EVENT = 'webkitTransitionEnd',
HORIZONTAL = 'horizontal',
//粘性
VISCOSITY = 5,
//触摸点偏移量
TOUCH_THRESHOLD = 5
var _window = window,
_prototype = Swiper.prototype
//获取触摸点
var getCoords = function(event) {
var touches = event.touches,
data = touches && touches.length ? touches : event.changedTouches
return {
x: isTouch ? data[0].pageX : event.pageX,
y: isTouch ? data[0].pageY : event.pageY
}
}
//获取偏移
var getDistance = function(event, startCoords) {
var coords = getCoords(event),
x = coords.x - startCoords.x,
y = coords.y - startCoords.y
return {
distanceX: x,
distanceY: y
}
}
//获取位置与索引
var getCoordinates = function(swiper, distanceX, distanceY) {
var element = swiper._ui.slides[0],
w = element.offsetWidth,
h = element.offsetHeight,
l = swiper._ui.slidesLength,
index = swiper._ui.active,
active = index,
threshold = swiper.options.threshold,
reach,
_distanceY = Math.abs(distanceY),
_distanceX = Math.abs(distanceX)
if (swiper.options.direction === HORIZONTAL) {
//达到门槛
reach = threshold < _distanceX
//横向
if (distanceX > 0 && index == 0) {
//最左侧
distanceX = distanceX / VISCOSITY
index = 0
} else if (distanceX < 0 && index == l - 1) {
//最右侧
distanceX = distanceX / VISCOSITY
index = l - 1
} else if (reach) {
//达到最小偏移量
//取整
var s = Math.round(_distanceX / w)
s = s == 0 ? 1 : s
//向右或者向左
index = distanceX > 0 ? index - s : index + s
}
return {
x: distanceX + (-w * active),
y: 0,
index: index,
isDefaultPrevented: !(!reach && _distanceX < _distanceY && TOUCH_THRESHOLD < _distanceY)
}
} else {
//达到门槛
reach = threshold < _distanceY
//垂直
if (distanceY > 0 && index == 0) {
//最上
distanceY = distanceY / VISCOSITY
index = 0
} else if (distanceY < 0 && index == l - 1) {
//最下
distanceY = distanceY / VISCOSITY
index = l - 1
} else if (reach) {
//达到最小偏移
var s = Math.round(_distanceY / h)
s = s == 0 ? 1 : s
index = distanceY > 0 ? index - s : index + s
}
return {
x: 0,
y: distanceY + (-h * active),
index: index,
isDefaultPrevented: true
}
}
}
var returnFalse = function() {
return false
}
//添加重复子节点
var appendCloneChildren = function(swiper) {
if (swiper.options.infinite) {
var $slides = swiper._ui.slides,
first = $slides[0],
last = $slides[swiper._ui.slidesLength - 1],
parentElement = first.parentElement
parentElement.insertBefore(last.cloneNode(true), first)
parentElement.appendChild(first.cloneNode(true))
swiper._ui.slidesLength += 2
}
}
//重置索引值
var resetSlideIndex = function(swiper) {
var index = swiper._ui.active,
length = swiper._ui.slidesLength
if (swiper.options.infinite) {
if (index === length - 1) {
swiper.slide(1, 0)
}
if (index === 0) {
swiper.slide(length - 2, 0)
}
}
}
//设置偏移量
var setTranslate = function($element, x, y) {
core.isNumber(x) && (x += 'px')
core.isNumber(y) && (y += 'px')
var t = 'translate3d(' + x + ',' + y + ',0)'
$element.css({
'-webkit-transform': t,
'transform': t
})
}
//设置偏移速度
var setDuration = function($element, duration) {
core.isNumber(duration) && (duration += 'ms')
$element.css({
'-webkit-transition-duration': duration,
'transition-duration': duration
})
}
var getActualIndex = function(index, length) {
return index < 0 ? 0 : index >= length ? length - 1 : index
}
//设置容器偏移量
var setContainerTranslate = function(swiper, x, y, duration) {
var $element = swiper.$element
duration = duration || 0
setDuration($element, duration)
setTranslate($element, x, y)
}
//添加选中类
var setActiveClass = function(swiper, index) {
var options = swiper.options,
slideActiveClass = options.slideActiveClass,
paginationActiveClass = options.paginationActiveClass
//添加选中的class
swiper._ui.slides.removeClass(slideActiveClass).eq(index).addClass(slideActiveClass)
swiper._ui.paginations.removeClass(paginationActiveClass).eq(index).addClass(paginationActiveClass)
}
//监听slide完成事件
var bindTransitionEndEvent = function(swiper) {
var $element = swiper.$element
var handler = function() {
var options = swiper.options,
callback = options.slide,
index = swiper._ui.active
resetSlideIndex(swiper)
//计算出真实索引值
options.infinite && (index = swiper._ui.active - 1)
callback && callback.call(swiper, index)
}
//duration == 0 无法触发
//translate 值未改变也无法触发
$element.on(TRANSITIONEND_EVENT, handler).on(WEBKIT_TRANSITIONEND_EVENT, handler)
}
//监听横竖屏切换
var bindOrientationChangeEvent = function(swiper) {
var timer
function handler() {
timer && clearTimeout(timer)
timer = setTimeout(function() {
swiper.slide(swiper._ui.active)
}, 200)
}
$(_window).on(RESIZE_EVENT, handler)
}
//绑定事件
var bindEvents = function(swiper) {
var flag = false,
startCoords
var start = function(event) {
stopAuto(swiper)
flag = true
event = event.originalEvent || event
resetSlideIndex(swiper)
startCoords = getCoords(event)
setDuration(swiper.$element, null)
},
move = function(event) {
if (!flag) return
event = event.originalEvent || event
var distance = getDistance(event, startCoords),
coordinates = getCoordinates(swiper, distance.distanceX, distance.distanceY)
coordinates.isDefaultPrevented && (event.preventDefault(),setTranslate(swiper.$element, coordinates.x, coordinates.y))
},
end = function(event) {
if (!flag) return
flag = false
event = event.originalEvent || event
var distance = getDistance(event, startCoords),
index = getCoordinates(swiper, distance.distanceX, distance.distanceY).index
swiper.slide(index)
beginAuto(swiper)
}
//监听横竖屏
bindOrientationChangeEvent(swiper)
//触发回调函数
bindTransitionEndEvent(swiper)
swiper.$element.on(START_EVENT, start)
swiper.$element.on(MOVE_EVENT, move)
swiper.$element.on(END_EVENT, end)
swiper.$element[0].onselectstart = returnFalse
swiper.$element[0].ondragstart = returnFalse
}
//偏移到指定的位置
var slideTo = function(swiper, index, duration) {
var element = swiper._ui.slides[0]
//由于移动端浏览器 transition 动画不支持百分比,所以采用像素值
if (swiper.options.direction === HORIZONTAL) {
//横向
var w = element.offsetWidth
setContainerTranslate(swiper, -index * w, 0, duration)
} else {
//垂直
var h = element.offsetHeight
setContainerTranslate(swiper, 0, -index * h, duration)
}
}
// 开始自动切换
var beginAuto = function(swiper){
var options = swiper.options,
_ui = swiper._ui,
auto = options.auto
auto && (swiper._timer = setInterval(function(){
// 由于一些特殊设计
// 对非循环滚动采用自动计算索引值的方式
options.infinite ? swiper.next() : swiper.slide( (_ui.active + 1) % _ui.slidesLength )
}, auto))
}
// 停止自动切换
var stopAuto = function(swiper){
var timer = swiper._timer
timer && clearInterval(timer)
}
//初始化
var init = function(swiper) {
var options = swiper.options
appendCloneChildren(swiper)
//设置默认样式
swiper.$element.css(options.style.swiper)
var initialSlide = options.initialSlide || 0
options.infinite && (initialSlide = initialSlide + 1)
//滚动到默认位置
swiper.slide(initialSlide, 0)
beginAuto(swiper)
//绑定事件
bindEvents(swiper)
}
/**
* ## Swiper.prototype.defaults
*
* `Swiper`默认配置项。
*
* 配置项说明:
*
* * `direction`: `String` 切换方向。horizontal :横向, vertical :纵向。
*
* * `threshold`: `Number` 最小触发距离。手指移动距离必须超过`threshold`才允许切换。
*
* * `duration`: `Number` 切换速度。
*
* * `auto`: `Number` 自动切换速度。0表示不自动切换,默认为0 。
*
* * `infinite`: `Boolean` 是否循环滚动 true :循环 false :不循环。
*
* * `initialSlide`: `Number` 初始化滚动位置。
*
* * `slideSelector`: `Selector` 滚动元素。
*
* * `slideActiveClass`: `String` 滚动元素选中时的类名。
*
* * `paginationSelector`: `Selector` 缩略图或icon。
*
* * `paginationActiveClass`: `String` 缩略图或icon选中时的类名。
*
* * `slide`: `Function` 切换回调函数。
*/
_prototype.defaults = {
//vertical
direction: HORIZONTAL,
threshold: 50,
duration: 300,
infinite: false,
initialSlide: 0,
slideSelector: '',
slideActiveClass: '',
paginationSelector: '',
paginationActiveClass: '',
slide: null,
style: {
swiper: {
'-webkit-transition-property': '-webkit-transform',
'transition-property': 'transform',
'-webkit-transition-timing-function': 'ease-out',
'transition-timing-function': 'ease-out'
}
}
}
/**
* ## Swiper.prototype.slide
*
* 滚动到指定索引值位置
*
* @param {index} index 滚动索引值
* @param {duration} duration 滚动速度,默认配置的`duration`。
* @return {instance} 当前实例
*/
_prototype.slide = function(index, duration) {
var options = this.options
//如果speed为空,则取默认值
duration = duration == null ? options.duration : duration
//取出实际的索引值,保存当前索引值
this._ui.active = index = getActualIndex(index, this._ui.slidesLength)
//通过索引值设置偏移
slideTo(this, index, duration)
//设置选中状态Class
setActiveClass(this, options.infinite ? index - 1 : index)
return this
}
/**
* ## Swiper.prototype.next
*
* 切换到下一个
*
* @param {duration} duration 滚动速度,默认配置的`duration`。
* @return {instance} 当前实例
*/
_prototype.next = function(duration) {
return this.slide(this._ui.active + 1, duration)
}
/**
* ## Swiper.prototype.prev
*
* 切换到上一个
*
* @param {duration} duration 滚动速度,默认配置的`duration`。
* @return {instance} 当前实例
*/
_prototype.prev = function(duration) {
return this.slide(this._ui.active - 1, duration)
}
module.exports = Swiper
/***/ }),
/* 23 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # DatePicker
*
* 时间选择器。格式化参照 [`DateHelper`](./date.js.html)
*
*/
/**
* @require [core](./core.js.html)
* @require [Lite](./lite.js.html)
* @require [detect](./detect.js.html)
* @require [Popup](./popup.js.html)
* @require [DateHelper](./date.js.html)
*/
var core = __webpack_require__(9),
$ = __webpack_require__(7),
os = __webpack_require__(10),
Popup = __webpack_require__(24),
template = __webpack_require__(26);
__webpack_require__(11)
var HEIGHT_UNIT = 54,
DURATION = 200,
COLUMN_SELECTOR = '.kub-datepicker-column',
POPUP_CLASS_NAME = 'kub-datepicker-popup',
DIALOG_BUTTON_SELECTOR = '.J_dialogButton',
COLUMN_ITEM_SELECTOR = 'li',
COLUMN_CONTAINER_SELECTOR = 'ul'
var $document = $(document),
isTouch = os.mobile
var START_EVENT = isTouch ? 'touchstart' : 'mousedown',
MOVE_EVENT = isTouch ? 'touchmove' : 'mousemove',
END_EVENT = isTouch ? 'touchend' : 'mouseup',
EVENT_NAME = 'click'
//渲染对话框
var render = function(datepicker) {
var options = datepicker.options,
html = template(options)
var popup = datepicker.$element[0].popup = new Popup({
title: options.title,
message: html,
className: options.className || '' + ' ' + POPUP_CLASS_NAME,
closeHandler:function(){
this.hide()
return false
}
})
var handlers = [
function(e) {
var cancel = options.cancel
cancel ? cancel.call(this, e, datepicker) : popup.hide()
},
function(e) {
var confirm = options.confirm,
formatDate = datepicker.getDate().format(options.format)
confirm ? confirm.call(this, e, datepicker, formatDate) : function() {
datepicker.$element[0].value = formatDate
popup.hide()
}()
}
]
//注册按钮事件
popup.$element.find(DIALOG_BUTTON_SELECTOR).on(EVENT_NAME, function(e) {
var index = parseInt($(this).attr('data-index')),
handler = handlers[index]
handler && handler.call(this, e, popup)
})
}
/**
* ## DatePicker Constructor
*
* `DatePicker` 构造函数。
*
* 使用:
* ```js
* //采用默认的format yyyy-MM-dd
* var datepicker = new Kub.DatePicker($('#J_datepicker'))
*
* //采用默认的format yyyy-MM-dd
* //可配置title 与 本地化
* var datepicker1 = new Kub.DatePicker($('#J_datepicker1'),{
* title:'Date Picker',
* locale:'en'
* })
*
* //自定义format
* var datepicker2 = new Kub.DatePicker($('#J_datepicker2'),{
* title:'选择时间',
* format:'yyyy-MM-dd,HH:mm:ss',
* confirm:function(e,datepicker,formatDate){
* //格式化后的 formatDate
* //手动关闭选择器
* datepicker.hide()
* }
* })
* ```
*/
var _prototype
//获取触摸点
var getCoords = function(event) {
var touches = event.touches,
data = touches && touches.length ? touches : event.changedTouches
return {
x: isTouch ? data[0].pageX : event.pageX,
y: isTouch ? data[0].pageY : event.pageY
}
}
//获取偏移
var getDistance = function(event, startCoords) {
var coords = getCoords(event),
x = coords.x - startCoords.x,
y = coords.y - startCoords.y
return {
distanceX: x,
distanceY: y
}
}
var returnFalse = function() {
return false
}
//获取当月最大天数
var getDays = function(year, month) {
return new Date(year, month + 1, 0).getDate()
}
//根据偏移量算出索引值
var getIndexByDistance = function(y, max) {
//去掉空白的两行
max = max - 5
y = y > 0 ? 0 : y
var index = Math.round(Math.abs(y) / HEIGHT_UNIT)
return index > max ? max : index
}
//设置偏移速度
var setDuration = function($this, duration) {
var $container = $this[0].$container,
transition = 'transform ' + duration + 'ms ease-out',
webkiTransition = '-webkit-transform ' + duration + 'ms ease-out'
$container.css({
'-webkit-transition': duration != null ? webkiTransition : duration,
'transition': duration != null ? transition : duration
})
}
//设置偏移速度
var setTranslate = function($this, x, y) {
var $container = $this[0].$container,
t
core.isNumber(x) && (x += 'px')
core.isNumber(y) && (y += 'px')
t = 'translate3d(' + x + ',' + y + ',0)'
!$container && ($container = $this[0].$container = $this.find(COLUMN_CONTAINER_SELECTOR))
$container.css({
'-webkit-transform': t,
'transform': t
})
}
//设置时间选择器中某一列的值,可设置年、月、日、时、分、秒的值
var setValue = function(datepicker, name, value) {
var $this = datepicker._ui[name],
index, $item
if ($this && ($item = $this.find(COLUMN_ITEM_SELECTOR + '[data-value="' + value + '"]')).length) {
index = parseInt($item.attr('data-index'))
$this[0].index = index
setTranslate($this, 0, -index * HEIGHT_UNIT)
}
}
//获取时间选择器中某一列的值,可获取年、月、日、时、分、秒的值
var getValue = function(datepicker, name) {
var $this = datepicker._ui[name],
$items, index, value
if ($this) {
$items = $this.find(COLUMN_ITEM_SELECTOR)
index = $this[0].index+2
value = parseInt($items.eq(index).attr('data-value'))
}
return value ? value : 0
}
//移除不需要的列
var removeColumns = function(format, ui) {
format.indexOf('y') === -1 && (ui.year.remove(), ui.year = null)
format.indexOf('M') === -1 && (ui.month.remove(), ui.month = null)
format.indexOf('d') === -1 && (ui.day.remove(), ui.day = null)
format.indexOf('H') === -1 && (ui.hour.remove(), ui.hour = null)
format.indexOf('m') === -1 && (ui.minute.remove(), ui.minute = null)
format.indexOf('s') === -1 && (ui.second.remove(), ui.second = null)
}
//重置每月最大天数
var setActualDays = function(datepicker, year, month) {
var days = getDays(year, month),
day = getValue(datepicker, 'day')
days < day && setValue(datepicker, 'day', days)
}
//绑定输入框聚焦事件
var bindInputFocusEvent = function(datepicker) {
datepicker.$element.on(EVENT_NAME, function(event) {
//使输入框失去焦点
datepicker.$element[0].blur()
datepicker.show()
event.preventDefault()
})
}
//绑定事件
var bindEvents = function(datepicker) {
var flag = false,
$activeElement,
$element = datepicker.$element[0].popup.$element
var start = function(event) {
flag = true
event = event.originalEvent || event
this.startCoords = getCoords(event)
$activeElement = $(this)
setDuration($activeElement, null)
},
move = function(event) {
if (!flag) return
event = event.originalEvent || event
var distance = getDistance(event, $activeElement[0].startCoords)
setTranslate($activeElement, 0, distance.distanceY - HEIGHT_UNIT * $activeElement[0].index)
event.preventDefault()
},
end = function(event) {
if (!flag) return
flag = false
event = event.originalEvent || event
var distance = getDistance(event, $activeElement[0].startCoords),
max = $activeElement.find(COLUMN_ITEM_SELECTOR).length,
index = getIndexByDistance(distance.distanceY - HEIGHT_UNIT * $activeElement[0].index, max)
$activeElement[0].index = Math.abs(index)
//验证是否存在31,30,29天
setActualDays(datepicker, getValue(datepicker, 'year'), getValue(datepicker, 'month'))
setDuration($activeElement, DURATION)
setTranslate($activeElement, 0, -HEIGHT_UNIT * $activeElement[0].index)
}
datepicker._ui.columns.each(function() {
$(this).on(START_EVENT, function() {
start.apply(this, arguments)
})
this.onselectstart = returnFalse
this.ondragstart = returnFalse
})
$element.on(MOVE_EVENT, move)
$element.on(END_EVENT, end)
bindInputFocusEvent(datepicker)
}
function init(datepicker) {
var options = datepicker.options,
$element,
ui
//创建对话框
render(datepicker)
$element = datepicker.$element[0].popup.$element
//缓存dom
ui = datepicker._ui = {
year: $element.find('.year'),
month: $element.find('.month'),
day: $element.find('.day'),
hour: $element.find('.hour'),
minute: $element.find('.minute'),
second: $element.find('.second')
}
ui.columns = $element.find(COLUMN_SELECTOR)
//设置块高度
HEIGHT_UNIT = ui.columns.find(COLUMN_ITEM_SELECTOR)[0].offsetHeight || HEIGHT_UNIT
//隐藏对话框
datepicker.hide()
//移除
removeColumns(options.format, ui)
//设置本地化
$element.addClass('kub-datepicker-' + options.locale)
//设置默认时间
datepicker.setDate(options.date)
//绑定事件
bindEvents(datepicker)
}
function DatePicker(element, options) {
this.$element = $(element)
this.options = core.extend({}, _prototype.defaults, options || {})
init(this)
}
_prototype = DatePicker.prototype
/**
* ## DatePicker.prototype.defaults
*
* 默认配置项
*
* 配置项说明:
*
* * `locale`: `String` 本地化。本地化采用CSS实现。
*
* * `title`: `String` 时间选择器弹窗名称。
*
* * `confirmText`: `String` 确认按钮名称。
*
* * `confirm`: `Function` 单击确认按钮时触发的事件。
*
* > 如果未传递,单击时会默认关闭弹窗,并对输入框赋值。
* >
* > 如果传递,需调用`datepicker.close()`手动关闭弹窗,并且需要对输入框赋值。
*
* * `cancelText`: `String` 取消按钮名称。
*
* * `cancel`: `Function` 单击取消按钮时触发的事件。
*
* > 如果未传递,单击时会默认关闭弹窗。
* >
* > 如果传递,需调用`datepicker.close()`关闭弹窗。
*
* * `format`: `String` 日期格式。
*
* * `className`: `String` 弹窗类名,修改时需加上`kub-datepicker-popup`默认类名。
*
* * `date`: `Date` 默认显示时间。
*
* * `yearRange`: `Array` 年份显示区间。
*/
_prototype.defaults = {
locale: 'zh',
title: '',
confirmText: '确定',
confirm: null,
cancelText: '取消',
cancel: null,
format: 'yyyy-MM-dd',
className: '',
date: new Date(),
yearRange: [1970, 2050]
}
/**
* ## DatePicker.prototype.setDate
*
* 设置时间选择器时间。
*
* @param {Date} date 时间
* @return {instance} 当前实例
*/
_prototype.setDate = function(date) {
var self = this,
year = date.getFullYear(),
month = date.getMonth()
setValue(self, 'year', year)
setValue(self, 'month', month)
setValue(self, 'day', date.getDate())
setValue(self, 'hour', date.getHours())
setValue(self, 'minute', date.getMinutes())
setValue(self, 'second', date.getSeconds())
return self
}
/**
* ## DatePicker.prototype.getDate
*
* 获取时间选择器选择的时间。
*
* @return {Date} 获取到的时间
*/
_prototype.getDate = function() {
var values = {
year: getValue(this, 'year'),
month: getValue(this, 'month'),
day: getValue(this, 'day'),
hour: getValue(this, 'hour'),
minute: getValue(this, 'minute'),
second: getValue(this, 'second')
}
return new Date(values.year, values.month, values.day, values.hour, values.minute, values.second)
}
/**
* ## DatePicker.prototype.close
*
* 关闭时间选择器。
*
* @return {instance} 当前实例
*/
_prototype.close = function() {
this.$element[0].popup.close()
return this
}
/**
* ## DatePicker.prototype.show
*
* 显示时间选择器。
*
* @return {instance} 当前实例
*/
_prototype.show = function() {
this.$element[0].popup.show()
return this
}
/**
* ## DatePicker.prototype.hide
*
* 隐藏时间选择器。
*
* @return {instance} 当前实例
*/
_prototype.hide = function() {
this.$element[0].popup.hide()
return this
}
module.exports = DatePicker
/***/ }),
/* 24 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # Popup
*
* 对话框.
*/
/**
* @require [core](./core.js.html)
* @require [Lite](./lite.js.html)
*/
var core = __webpack_require__(9),
$ = __webpack_require__(7),
template = __webpack_require__(25)
/**
* ## Popup Constructor
*
* Popup 构造函数。
*
* 使用:
* ```js
* var popup = new Kub.Popup({
* message:'popup dialog'
* })
* ```
*/
function Popup(options) {
this.options = core.extend({}, _prototype.defaults, options || {})
init(this)
}
var ANIMATION_CLASS = 'kub-popup-animation',
EVENT_NAME = 'click',
POPUP_SELECTOR = '.J_popup'
var $body
var _prototype = Popup.prototype
var render = function(popup,data) {
var html = template(data)
popup.$element = $(html).appendTo($body)
return this
}
var bindEvents = function(popup){
popup.$element.on(EVENT_NAME, function(e) {
if($(e.target).closest(POPUP_SELECTOR).length){
return
}
popup.close()
})
}
var init = function(popup) {
!$body && ($body = $(document.body))
//渲染数据
render(popup, popup.options)
bindEvents(popup)
popup.show()
}
/**
* ## Popup.prototype.defaults
*
* 默认配置项。
*
* 配置项说明:
*
* * `message` : `String` 弹窗内容。
*
* * `className` : `String` 弹窗类名。
*
*/
_prototype.defaults = {
message: '',
className: '',
duration:350
}
/**
* ## Popup.prototype.show
*
* 显示弹窗。
* @return {instance} 返回当前实例
*/
_prototype.show = function() {
clearTimeout(this._timer)
this.$element.show().addClass(ANIMATION_CLASS)
return this
}
/**
* ## Popup.prototype.hide
*
* 隐藏弹窗。
* @return {instance} 返回当前实例
*/
_prototype.hide = function(callback) {
var self = this,
$element = self.$element
function handler(){
$element.hide()
callback && callback()
}
$element.removeClass(ANIMATION_CLASS)
clearTimeout(self._timer)
self._timer = setTimeout(function(){
handler()
},self.options.duration)
return self
}
/**
* ## Popup.prototype.close
*
* 关闭弹窗。
* @return {instance} 返回当前实例
*/
_prototype.close = function() {
var self = this,
opts = self.options
if (opts.closeHandler && opts.closeHandler.call(self) === false) {
return self
}
self.hide(function(){
self.$element.remove()
})
return self
}
module.exports = Popup
/***/ }),
/* 25 */
/***/ (function(module, exports) {
module.exports = function(data){
var __t,__p='',__j=Array.prototype.join,print=function(){__p+=__j.call(arguments,'');};
__p+='<div class="kub-dialog-modal kub-popup-modal '+
((__t=( data.className))==null?'':__t)+
'"><div class="kub-popup J_popup"> '+
((__t=( data.message))==null?'':__t)+
' </div></div>';
return __p;
};
/***/ }),
/* 26 */
/***/ (function(module, exports) {
module.exports = function(data){
var __t,__p='',__j=Array.prototype.join,print=function(){__p+=__j.call(arguments,'');};
__p+='<div class="kub-datepicker-header"><button class="kub-datepicker-button J_dialogButton" data-index="0"> '+
((__t=( data.cancelText))==null?'':__t)+
' </button><div class="kub-datepicker-header-text">'+
((__t=( data.title))==null?'':__t)+
'</div><button class="kub-datepicker-button J_dialogButton" data-index="1"> '+
((__t=( data.confirmText))==null?'':__t)+
' </button></div><div class="kub-datepicker"><div class="kub-datepicker-column year" data-type="year"><ul><li></li><li></li> ';
for(var i=data.yearRange[0],j=0;i<=data.yearRange[1];i++,j++){
__p+=' <li data-value="'+
((__t=( i))==null?'':__t)+
'" data-index="'+
((__t=( j))==null?'':__t)+
'"> '+
((__t=( i ))==null?'':__t)+
' </li> ';
}
__p+=' <li></li><li></li></ul></div><div class="kub-datepicker-column month" data-type="month"><ul><li></li><li></li> ';
for(var i=1 ;i<= 12; i++){
__p+=' <li data-value="'+
((__t=( i-1))==null?'':__t)+
'" data-index="'+
((__t=( i-1))==null?'':__t)+
'"> '+
((__t=( ( i < 10 ? ( "0" + i) : i)))==null?'':__t)+
' </li> ';
}
__p+=' <li></li><li></li></ul></div><div class="kub-datepicker-column day" data-type="day"><ul><li></li><li></li> ';
for(var i=1 ;i<=31;i++){
__p+=' <li data-value="'+
((__t=( i))==null?'':__t)+
'" data-index="'+
((__t=( i-1))==null?'':__t)+
'"> '+
((__t=(( i < 10 ? ( "0" + i) : i)))==null?'':__t)+
' </li> ';
}
__p+=' <li></li><li></li></ul></div><div class="kub-datepicker-column hour" data-type="hour"><ul><li></li><li></li> ';
for(var i=0 ;i<=23;i++){
__p+=' <li data-value="'+
((__t=( i))==null?'':__t)+
'" data-index="'+
((__t=( i))==null?'':__t)+
'"> '+
((__t=(( i < 10 ? ( "0" + i) : i)))==null?'':__t)+
' </li> ';
}
__p+=' <li></li><li></li></ul></div><div class="kub-datepicker-column minute" data-type="minute"><ul><li></li><li></li> ';
for(var i=0 ;i<=59;i++){
__p+=' <li data-value="'+
((__t=( i))==null?'':__t)+
'" data-index="'+
((__t=( i))==null?'':__t)+
'"> '+
((__t=( ( i < 10 ? ( "0" + i) : i)))==null?'':__t)+
' </li> ';
}
__p+=' <li></li><li></li></ul></div><div class="kub-datepicker-column second" data-type="second"><ul><li></li><li></li> ';
for(var i=0 ;i<=59;i++){
__p+=' <li data-value="'+
((__t=( i))==null?'':__t)+
'" data-index="'+
((__t=( i))==null?'':__t)+
'"> '+
((__t=( ( i < 10 ? ( "0" + i) : i)))==null?'':__t)+
' </li> ';
}
__p+=' <li></li><li></li></ul></div><div class="kub-datepicker-overlay"></div></div>';
return __p;
};
/***/ }),
/* 27 */
/***/ (function(module, exports, __webpack_require__) {
/**
* # Touch
*
* 移动端事件组件。
*
* 支持的事件包含:
*
* `tap` `longtap`
*
* `panstart` `panmove` `panup` `pandown` `panleft` `panright` `panend`
*
* `swipeleft` `swiperight` `swipeup` `swipedown`
*
*/
/**
* @require [polyfill](./polyfill.js.html)
*/
__webpack_require__(8)
var MOBILE_REGEXP = /mobile|tablet|ip(ad|hone|od)|android/i
var _window = window,
isTouch = 'ontouchstart' in _window && MOBILE_REGEXP.test(navigator.userAgent)
var EVENTS_METHODS = [
'preventDefault',
'stopImmediatePropagation',
'stopPropagation'
]
var SWIPE_THRESHOLD = 10,
SWIPE_VELOCITY = 0.25,
SWIPE_MAX_MOVEMENT = 6,
TAP_TIMEOUT = 200,
TAP_THRESHOLD = 9,
LONGTAP_TIMEOUT = 500,
START_EVENT = isTouch ? 'touchstart' : 'mousedown',
MOVE_EVENT = isTouch ? 'touchmove' : 'mousemove',
END_EVENT = isTouch ? 'touchend' : 'mouseup',
DIRECTION_ANGLE = 25,
DIRECTIONS = ['up', 'right', 'down', 'left'],
SWIPE_EVENT = 'swipe',
PAN_EVENT = 'pan',
PAN_START_EVENT = 'panstart',
PAN_MOVE_EVENT = 'panmove',
PAN_END_EVENT = 'panend',
TAP_EVENT = 'tap',
LONGTAP_EVENT = 'longtap'
// 获取位移量
var distance = function(p1, p2) {
return Math.round(Math.sqrt(Math.pow((p1.x - p2.x), 2) + Math.pow((p1.y - p2.y), 2)))
}
// 获取角度,通过获取角度从而获取方向
var angle = function(p1, p2) {
var d = Math.abs(p2.x - p1.x)
return Math.round(Math.acos(d / Math.sqrt(Math.pow(d, 2) + Math.pow(p2.y - p1.y, 2))) * 57.3)
}
// 获取方向
var direction = function(p1, p2) {
return (angle(p1, p2) > DIRECTION_ANGLE) ? ((p1.y < p2.y) ? 2 : 0) : ((p1.x < p2.x) ? 1 : 3)
}
// 如果触摸点位移大于 SWIPE_THRESHOLD 而且速度大于 SWIPE_VELOCITY
var matchSwipe = function(threshold, interval) {
return threshold != null && threshold > SWIPE_THRESHOLD && threshold / interval > SWIPE_VELOCITY
}
// 如果触摸点位置大于 TAP_THRESHOLD 而且间隔时间小于 TAP_TIMEOUT
var matchTap = function(threshold, interval) {
return threshold != null && threshold < TAP_THRESHOLD && interval < TAP_TIMEOUT
}
// 获取触摸点数据
var getCoords = function(event) {
var touches = event.touches,
data = touches && touches.length ? touches : event.changedTouches
return {
x: isTouch ? data[0].clientX : event.clientX,
y: isTouch ? data[0].clientY : event.clientY,
e: isTouch ? data[0].target : event.target
}
}
// 获取事件位置数据
var getEventDetail = function(coords) {
return {
x: coords.x,
y: coords.y
}
}
// 获取偏移值与时间间隔
var getThresholdAndInterval = function(p1,p2){
return {
threshold : p1 && p2 && distance(p1, p2),
interval : p1 && p2 && (p2.t.getTime() - p1.t.getTime())
}
}
// 触发事件
var trigger = function(element, type, originalEvent, detail) {
var event = new _window.CustomEvent(type, {
detail: detail,
bubbles: true,
cancelable: true
})
// 存储原事件对象
event.originalEvent = originalEvent
EVENTS_METHODS.forEach(function(name){
event[name] = function(){
originalEvent[name].apply(originalEvent,arguments)
}
})
element && element.dispatchEvent && element.dispatchEvent(event)
}
var on = function(element, type, handler) {
element.addEventListener(type, handler, false)
}
var clearTime = function(timer) {
timer && clearTimeout(timer)
timer = null
}
var findMatchedDirection = function(actions){
var index = 0, max = actions[index]
actions.forEach(function(value,i){
value > max && (max = value, index = i)
})
return index
}
/**
* ## Touch Constructor
*
* `Touch`类。
*
* 使用方法:
* ```js
*
* new Kub.Touch(element)
*
* // swipeleft 事件,支持事件代理
* element.addEventListener('swipeleft','div',function(){
* //do something
* })
*
* // tap 事件
* element.addEventListener('tap',function(){
* //do something
* })
*
* ```
*/
function Touch(element) {
var moveFlag = false,
target,
p1,
p2,
longTapTimer,
cancelTap = false,
actions,
actionsLength
on(element, START_EVENT, function(event) {
var coords = getCoords(event)
p1 = coords
p1.t = new Date()
p2 = p1
actions = [0,0,0,0]
actionsLength = 0
cancelTap = false
target = coords.e
//触发 longtap 事件
isTouch && (longTapTimer = setTimeout(function() {
trigger(target, LONGTAP_EVENT, event)
}, LONGTAP_TIMEOUT))
})
on(element, MOVE_EVENT, function(event) {
if(!target){
return
}
var coords = getCoords(event),
detail = getEventDetail(coords),
thresholdAndInterval,
direct
p2 = coords
p2.t = new Date()
thresholdAndInterval = getThresholdAndInterval(p1,p2)
// 如果触摸点不符合 longtap 触发条件,则取消长按事件
if(!cancelTap && !matchTap(thresholdAndInterval.threshold, thresholdAndInterval.interval)){
clearTime(longTapTimer)
cancelTap = true
}
//触发 panstart 事件
!moveFlag && trigger(target, PAN_START_EVENT, event, detail)
direct = direction(p1, p2)
// 取出前SWIPE_MAX_MOVEMENT移动记录
actionsLength < SWIPE_MAX_MOVEMENT && (actions[direct] += 1, actionsLength += 1)
//触发 pan['up', 'right', 'down', 'left'] 事件
trigger(target, PAN_EVENT + DIRECTIONS[direct], event, detail)
//触发 panmove 事件
trigger(target, PAN_MOVE_EVENT, event, detail)
moveFlag = true
})
on(element, END_EVENT, function(event) {
// 取消 longtap 事件定时器
clearTime(longTapTimer)
var coords = getCoords(event), thresholdAndInterval
p2 = coords
p2.t = new Date()
thresholdAndInterval = getThresholdAndInterval(p1,p2)
// 如果达到 swipe 事件条件
if (matchSwipe(thresholdAndInterval.threshold, thresholdAndInterval.interval)) {
//触发 swipe['up', 'right', 'down', 'left'] 事件
trigger(target, SWIPE_EVENT + DIRECTIONS[findMatchedDirection(actions)], event)
} else if (!cancelTap && isTouch && matchTap(thresholdAndInterval.threshold, thresholdAndInterval.interval)) {
// 触发 tap 事件
trigger(target, TAP_EVENT, event)
}
// 触发 panend 事件
target && moveFlag && trigger(target, PAN_END_EVENT, event, getEventDetail(coords))
target = null
moveFlag = false
})
}
module.exports = Touch
/***/ })
/******/ ]);<file_sep>module.exports = function(data){
var __t,__p='',__j=Array.prototype.join,print=function(){__p+=__j.call(arguments,'');};
__p+='<div class="kub-dialog-modal '+
((__t=( data.className))==null?'':__t)+
' ';
if( data.modal ){
__p+=' kub-modal ';
}
__p+='"><div class="kub-dialog-wrapper"><div class="kub-dialog-container"><div class="kub-dialog J_dialog"> ';
if(data.showHeader){
__p+=' <div class="kub-dialog-header"> '+
((__t=( data.title))==null?'':__t)+
' </div> ';
}
__p+=' <div class="kub-dialog-body"> '+
((__t=( data.message))==null?'':__t)+
' </div> ';
if(data.buttons && data.buttons.length){
__p+=' <div class="kub-dialog-footer"> ';
for (var i=0,j=data.buttons.length;i<j;i++){
__p+=' <button class="kub-dialog-button J_dialogButton '+
((__t=( data.buttons[i].className || ''))==null?'':__t)+
'" data-index="'+
((__t=( i))==null?'':__t)+
'"> '+
((__t=( data.buttons[i].text))==null?'':__t)+
' </button> ';
}
__p+=' </div> ';
}
__p+=' </div></div></div></div>';
return __p;
};<file_sep>/**
* # cookie
*
* 操作cookie方法,`expires` 如果为数字,单位为`毫秒`。
*
* 使用方法:
* ```js
* //取值
* var name = Kub.cookie('name')
*
* //设置值
* Kub.cookie('name','kub')
*
* //配置cookie相关属性
* Kub.cookie('name','kub',{
* domain:'.a.com'
*
* })
* ```
*
*/
var _document = document,
_encodeURIComponent = encodeURIComponent
function cookie(key, value, options) {
var days, time, result, decode
options = options || {}
if(!options.hasOwnProperty('path')){
options.path = '/'
}
// A key and value were given. Set cookie.
if (arguments.length > 1 && String(value) !== "[object Object]") {
// Enforce object
if (value === null || value === undefined) options.expires = -1
if (typeof options.expires === 'number') {
days = options.expires
time = options.expires = new Date()
time.setTime(time.getTime() + days)
}
value = String(value)
return (_document.cookie = [
_encodeURIComponent(key), '=',
options.raw ? value : _encodeURIComponent(value),
options.expires ? '; expires=' + options.expires.toUTCString() : '',
options.path ? '; path=' + options.path : '',
options.domain ? '; domain=' + options.domain : '',
options.secure ? '; secure' : ''
].join(''))
}
// Key and possibly options given, get cookie
options = value || {}
decode = options.raw ? function (s) { return s } : decodeURIComponent
return (result = new RegExp('(?:^|; )' + _encodeURIComponent(key) + '=([^;]*)').exec(_document.cookie)) ? decode(result[1]) : null
}
module.exports = cookie
| dd40fd0963d222c1bc8d06803ae91a9a63f57854 | [
"JavaScript",
"Markdown"
] | 20 | JavaScript | longjiarun/kubjs.git | cc065e9433a30c7810a38994ac3e8a9224b27a75 | acc977e3688334a3dfaa4c29a1fb7142aa0da9fe | |
refs/heads/master | <repo_name>Glydion/tetris1<file_sep>/main.cpp
//******************************************
// Tetris - v1.0.0
// By: <NAME>. (Glydion)
//
// Start Date: Sunday July 8, 2018
// Finish Date:
//******************************************
#include "main.h"
int main( int argc, char* argv[] )
{
// Create the game object
Game* game = new Game();
// Initialize and run the game
if( game->Init() )
{
game->Run();
}
// Clean up
delete game;
return 0;
}
<file_sep>/Tetrimino.cpp
#include "Tetrimino.h"
Tetrimino::Tetrimino(SDL_Renderer* renderer)
{
// Initialize data members
this->renderer = renderer;
// Initialize tile set spritesheet
SDL_Surface* surface = IMG_Load("images/tiles.png");
tileSet = SDL_CreateTextureFromSurface(renderer,surface);
SDL_FreeSurface(surface);
CreateShape();
}
Tetrimino::~Tetrimino()
{
}
void Tetrimino::CreateShape()
{
int n = 3;
//if(a[0].x == 0)
//{
for( int i = 0; i < 4; i++ )
{
a[i].x = figures[n][i] % 2;
a[i].y = figures[n][i] / 2;
}
//}
}
void Tetrimino::RotatePiece()
{
if(isRotating)
{
// Determine the center of rotation
Point p = a[1];
for(int i = 0; i < 4; i++)
{
int x = a[i].y - p.y;
int y = a[i].x - p.x;
a[i].x = p.x - x;
a[i].y = p.y + y;
}
}
}
void Tetrimino::MovePiece()
{
if(isMoving)
{
switch(moveDirection)
{
case -1:
{
for(int i = 0; i < 4; i++)
{
a[i].x -= moveDirection;
}
break;
}
case 1:
{
for(int i = 0; i < 4; i++)
{
a[i].x += moveDirection;
}
break;
}
case 2:
{
for(int i = 0; i < 4; i++)
{
a[i].y += 1;
}
}
}
}
}
void Tetrimino::HandleEvent(SDL_Event& e)
{
// If a key was pressed...
if(e.type == SDL_KEYDOWN)
{
// Check which key was pressed
switch(e.key.keysym.sym)
{
case SDLK_LEFT:
{
isMoving = true;
moveDirection = -1;
break;
}
case SDLK_RIGHT:
{
isMoving = true;
moveDirection = 1;
break;
}
case SDLK_DOWN:
{
isMoving = true;
moveDirection = 2;
break;
}
case SDLK_SPACE:
{
isRotating = true;
break;
}
}
}
// If a key was released...
else if(e.type == SDL_KEYUP)
{
switch(e.key.keysym.sym)
{
case SDLK_LEFT:
{
// Stop moving the Tetrimino left
isMoving = false;
moveDirection = 0;
break;
}
case SDLK_RIGHT:
{
// Stop moving the Tetrimino right
isMoving = false;
moveDirection = 0;
break;
}
case SDLK_DOWN:
{
isMoving = false;
moveDirection = 0;
break;
}
case SDLK_SPACE:
{
// Stop rotating the Tetrimino
isRotating = false;
break;
}
}
}
}
void Tetrimino::Update(float delta)
{
// Every frame, check to see whether
// to move or rotate the Tetrimino (or both)
this->RotatePiece();
this->MovePiece();
}
void Tetrimino::Render(float delta)
{
// Render all tiles of the tetrimino piece
for( int i = 0; i < 4; i++ )
{
SDL_Rect srcRect;
srcRect.x = 0;
srcRect.y = 0;
srcRect.w = CELL_WIDTH;
srcRect.h = CELL_HEIGHT;
// Set destination rectangle for each tile
SDL_Rect dstRect;
dstRect.x = a[i].x * CELL_WIDTH;
dstRect.y = a[i].y * CELL_HEIGHT;
dstRect.w = CELL_WIDTH;
dstRect.h = CELL_HEIGHT;
SDL_RenderCopy(renderer,tileSet,&srcRect,&dstRect);
}
}
<file_sep>/Game.h
#ifndef GAME_H_
#define GAME_H_
// Preprocessors
#include "SDL.h"
#include "SDL_image.h"
#include "SDL_ttf.h"
#include "SDL_mixer.h"
#include <iostream>
#include <sstream>
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <time.h>
#include <cmath>
#include <vector>
// Inclusions for game objects
#include "Tetrimino.h"
// Macros
#define FPS_DELAY 500
#define SCREEN_WIDTH 320
#define SCREEN_HEIGHT 480
#define GRID_WIDTH 10
#define GRID_HEIGHT 20
class Game
{
public:
Game();
~Game();
bool Init();
void Run();
private:
SDL_Window* window;
SDL_Renderer* renderer;
SDL_Texture* texture;
// Game objects
Tetrimino* testPiece;
// Audio objects
Mix_Music* gameMusic = NULL;
// Timing
unsigned int lastTick, fpsTick, fps, frameCount;
// Font objects
TTF_Font* fontScore;
std::stringstream sValue;
SDL_Texture* sTexture;
int sWidth, sHeight;
// Standard game functions
void Clean();
void Update(float delta);
void Render(float delta);
void NewGame();
};
#endif // GAME_H_
<file_sep>/Game.cpp
#include "Game.h"
Game::Game()
{
window = 0;
renderer = 0;
}
Game::~Game()
{
}
bool Game::Init()
{
// Initialize the SDL video and audio subsystems
SDL_Init(SDL_INIT_VIDEO | SDL_INIT_AUDIO);
// Create window
window = SDL_CreateWindow("Tetris v1.0", SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED,
SCREEN_WIDTH, SCREEN_HEIGHT, SDL_WINDOW_SHOWN | SDL_WINDOW_OPENGL );
if(!window)
{
std::cout << "Error creating window: " << SDL_GetError() << std::endl;
return false;
}
// Create renderer
renderer = SDL_CreateRenderer(window, -1, SDL_RENDERER_ACCELERATED | SDL_RENDERER_PRESENTVSYNC);
if(!renderer)
{
std::cout << "Error creating renderer: " << SDL_GetError() << std::endl;
return false;
}
// Enable TTF loading
TTF_Init();
// Load the fonts
fontScore = TTF_OpenFont("Munro.ttf", 20);
// Initialize SDL mixer
if( Mix_OpenAudio(44100, MIX_DEFAULT_FORMAT, 2, 2048) < 0 )
{
std::cout << "Error initializing SDL mixer: " << Mix_GetError() << std::endl;
return false;
}
// Load music
gameMusic = Mix_LoadMUS("sounds/tetris.mp3");
if( !gameMusic )
{
std::cout << "Error loading Tetris music: " << Mix_GetError() << std::endl;
return false;
}
// Load sounds
// Seed random number generation
srand(time(0));
// Initialize timing
lastTick = SDL_GetTicks();
fpsTick = lastTick;
fps = 0; // Set starting FPS value
frameCount = 0; // Set starting frame count
return true;
}
void Game::Clean()
{
// Clean resources
SDL_DestroyTexture(texture);
SDL_DestroyRenderer(renderer);
SDL_DestroyWindow(window);
Mix_FreeMusic(gameMusic);
gameMusic = NULL;
// Clean test data
SDL_DestroyTexture(sTexture);
}
void Game::Run()
{
// Create game objects
testPiece = new Tetrimino(renderer);
// Start the game music
Mix_PlayMusic(gameMusic,-1);
// Start a new game
NewGame();
// Main game loop
while(1)
{
// Event handler
SDL_Event e;
// If event is a QUIT event, stop the program
if(SDL_PollEvent(&e))
{
if(e.type == SDL_QUIT)
{
break;
}
// Handle input for the Tetrimino
testPiece->HandleEvent(e);
}
// Calculate delta and fps
unsigned int curTick = SDL_GetTicks();
float delta = (curTick - lastTick) / 1000.0f;
// Cap FPS delay to specific amount
if(curTick - fpsTick > FPS_DELAY)
{
fps = frameCount * (1000.0f / (curTick - fpsTick));
fpsTick = curTick;
frameCount = 0;
//std::cout << "FPS: " << fps << std::endl;
char buf[100];
snprintf(buf,100,"Tetris v1.0 (fps: %u)",fps);
SDL_SetWindowTitle(window,buf);
}
else
{
frameCount++;
}
lastTick = curTick;
// Update and render the game
Update(delta);
Render(delta);
}
delete testPiece;
Clean();
// Close the fonts
TTF_CloseFont(fontScore);
// Close all subsystems
TTF_Quit();
Mix_Quit();
SDL_Quit();
}
void Game::NewGame()
{
}
void Game::Update(float delta)
{
// Update the Tetrimino test piece
testPiece->Update(delta);
}
void Game::Render(float delta)
{
// Clear the renderer
SDL_RenderClear(renderer);
// Render the game objects
testPiece->Render(delta);
// Present the renderer to display
SDL_RenderPresent(renderer);
}
<file_sep>/Tetrimino.h
#ifndef TETRIMINO_H_INCLUDED
#define TETRIMINO_H_INCLUDED
#define CELL_WIDTH 18
#define CELL_HEIGHT 18
#define MOVE_DISTANCE 18
#include "SDL.h"
#include "SDL_image.h"
class Tetrimino
{
public:
Tetrimino(SDL_Renderer* renderer);
~Tetrimino();
// 2D array of pieces and their shapes
int figures[7][4] =
{
{1,3,5,7}, // I
{2,4,5,7}, // Z
{3,5,4,6}, // S
{3,5,4,7}, // T
{2,3,5,7}, // L
{3,5,7,6}, // J
{2,3,4,5}, // O
};
struct Point
{int x, y;} a[4], b[4];
void CreateShape();
void Update(float delta);
void Render(float delta);
void HandleEvent(SDL_Event& e);
void RotatePiece();
void MovePiece();
private:
SDL_Texture* tileSet;
SDL_Renderer* renderer;
bool isMoving;
bool isRotating;
int moveDirection;
};
#endif // TETRIMINO_H_INCLUDED
| 8a656f6e74327a488ac96f00f8a735c5f2c5fd6e | [
"C++"
] | 5 | C++ | Glydion/tetris1 | 65b03f5f6cf1412623bb6dbe8d87cb31a3d7b703 | 4abbcd03fba07d67933b8d15a8d52a2238e4a9e3 | |
refs/heads/master | <file_sep>package genomicsTest;
import java.util.List;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.ElementNotVisibleException;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebDriverException;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.interactions.Actions;
import org.testng.Assert;
import org.testng.annotations.BeforeSuite;
import org.testng.annotations.Test;
public class GenomicsTest2 {
public class GenomicsLoginTest {
public WebDriver driver;
private String baseUrl;
@BeforeSuite
public void setUp() throws Exception {
//driver = new FirefoxDriver();
System. setProperty("webdriver.chrome.driver", "C:/Drivers//chromedriver.exe");
driver = new ChromeDriver();
baseUrl = "https://cln-test-damsauto.gel.zone/labkey/";
driver.navigate().to(baseUrl);
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(30, TimeUnit.SECONDS);
}
//System.out.println("Title is Anil");
//System.out.println("Title is Anil");
/*@AfterSuite
public void tearDown() throws Exception {
driver.quit();
}*/
@Test
public void testGenomicsLogin() throws InterruptedException{
//Click on Singin
driver.findElement(By.className("labkey-nomenu-text-link")).click();
Thread.sleep(2000);
WebElement user=driver.findElement(By.id("email"));
user.sendKeys("<EMAIL>");
WebElement pwd=driver.findElement(By.id("password"));
pwd.sendKeys("<PASSWORD>");
driver.findElement(By.xpath("//a[@class='labkey-button signin-btn']/span")).click();
Thread.sleep(2000);
System.out.println("Title is " + driver.getTitle());
Assert.assertEquals("DAMS AUTO LabKey Server", driver.getTitle());
}
@Test(priority=1)
public void testGenomicsHomePage() throws InterruptedException{
// Click on Mercury
Thread.sleep(10000);
driver.findElement(By.xpath("//li[@id='projectBar']")).click();
Thread.sleep(10000);
driver.findElement(By.xpath("//div[@id='projectBar_menu']//a[text()='MeRCURy']")).click();
// Click on Rare Diseases
driver.findElement(By.linkText("Rare Diseases")).click();
Thread.sleep(2000);
// Click on v.13 Rare Diseases
driver.findElement(By.linkText("V1.3 Pedigree")).click();
//Click on Participant id
driver.findElement(By.xpath("//td[@title='participant_id']")).click();
Thread.sleep(5000);
//Click on filter
driver.findElement(By.xpath("//span[@id='menuitem-1133-textEl']")).click();
Thread.sleep(10000);
//Enter Filter Type
driver.findElement(By.xpath("//input[@id='value_1']")).sendKeys("800000981");
//Clcik on Ok
driver.findElement(By.xpath("//td[@class='x-btn-mc']//button")).click();
//Clcik on Participant id
Thread.sleep(10000);
driver.findElement(By.linkText("800000981")).click();
Thread.sleep(10000);
}
public void clickOnRect(String Element){
WebElement text=driver.findElement(By.xpath("//*[name()='text']"));
String iamgetesxt=text.getAttribute("x");
//String Y=text.getAttribute("Y");
String rect=driver.findElement(By.xpath("//*[name()='rect' and @class='pedigree-node-shadow']")).getAttribute("x");
WebElement rectclick=driver.findElement(By.xpath("//*[name()='rect' and @class='pedigree-node-shadow' and @x='image]"));
}
/* // Locate text and save in the WebElement instances.
List<WebElement>rectxyvalue=driver.findElements(By.xpath("//*[name()='rect']"));
for(WebElement r:rectxyvalue){
System.out.println("rect X value is:- " +r.getAttribute("x"));
System.out.println("rect y value is:- " +r.getAttribute("y"));
}
List<WebElement>textxyvalue=driver.findElements(By.xpath("//*[name()='text']"));
for(WebElement t:textxyvalue){
System.out.println("text X value is:- " +t.getAttribute("x"));
System.out.println("text y value is:- " +t.getAttribute("y"));
}
List<WebElement>text=driver.findElements(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='text']//*[name()='tspan']"));
for(WebElement t:text){
System.out.println("Name is " + t.getText());
if(t.getText().equals("RDPBMULTI1_4_FN RDPBMULTI1_4_SN")){
//select it
WebElement rect=driver.findElement(By.xpath("//*[name()='rect' and @class='pedigree-node-shadow']"));
System.out.println("rect X value is:- " +rect.getAttribute("x"));
if(rect.getAttribute("x").equals("644")){
Actions builder2 = new Actions(driver);
builder2.moveToElement(rect).click(rect);
builder2.perform();
} else
System.out.println("test is not matched");
}
}
String textyvalue=driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='text']//*[name()='tspan']")).getAttribute("y");
String rectxvalue=driver.findElement(By.xpath("//*[name()='rect' and @class='pedigree-hoverbox']")).getAttribute("x");
String rectyvalue=driver.findElement(By.xpath("//*[name()='rect' and @class='pedigree-hoverbox']")).getAttribute("y");
WebElement textxpath1=driver.findElement(By.xpath("//*[name()='text' and @x='684' and @y='616']//*[name()='tspan']"));
WebElement textxpath2=driver.findElement(By.xpath("//*[name()='text' and @x='444' and @y='72']//*[name()='tspan']"));
WebElement textxpath3=driver.findElement(By.xpath("//*[name()='text' and @x='300' and @y='344']//*[name()='tspan']"));
WebElement textxpath4=driver.findElement(By.xpath("//*[name()='text' and @x='60' and @y='344']//*[name()='tspan']"));
WebElement textxpath5=driver.findElement(By.xpath("//*[name()='text' and @x='1068' and @y='344']//*[name()='tspan']"));
WebElement recxpath=driver.findElement(By.xpath("//*[name()='text' and @x='1068' and @y='344']//*[name()='tspan']"));
public void pedigreeTest(String textxpath1, String recxpath){
}
try {
WebElement image3 =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='rect' and @x='644' and @y='504' and @class='pedigree-node-shadow' and @fill='#808080'][1]"));
Actions builder2 = new Actions(driver);
builder2.moveToElement(image3).click(image3);
builder2.perform();
} catch (WebDriverException e) {
System.out.println("Exception " + e);
try {
WebElement image3 =driver.findElement(By.xpath("//*[name()='rect' and @x='644' and @y='504'][3]"));
Actions builder2 = new Actions(driver);
builder2.moveToElement(image3).click(image3);
builder2.perform();
} catch (WebDriverException e1) {
System.out.println("Exception " + e1);
}
driver.findElement(By.xpath("//div[@class='menu-box']//span[@class='close-button']")).click();
try {
WebElement element =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='circle' and @cy='272' and @cx='300' and @fill='#808080' and @opacity='0.3'][1]"));
Actions builder = new Actions(driver);
Thread.sleep(10000);
builder.moveToElement(element).click(element);
builder.perform();
} catch (WebDriverException c) {
System.out.println("Exception " + c);
try {
WebElement image3 =driver.findElement(By.xpath("//*[name()='rect' and @x='644' and @y='504'][3]"));
Actions builder2 = new Actions(driver);
builder2.moveToElement(image3).click(image3);
builder2.perform();
} catch (WebDriverException e1) {
System.out.println("Exception " + e1);
}
//Click Pedigree Editor
//WebElement image3 =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='text' and @x='684'][1] and //*[name()='rect' and @x='644' and @y='504' and @class='pedigree-node-shadow' and @fill='#808080'][1]"));
//WebElement image3 =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='rect' and @x='644' and @y='504' and @class='pedigree-node-shadow' and @fill='#808080'][1]"));
Actions builder2 = new Actions(driver);
builder2.moveToElement(image3).click(image3);
builder2.perform();
Thread.sleep(10000);
//Close Popup
driver.findElement(By.xpath("//div[@class='menu-box']//span[@class='close-button']")).click();
//Click Pedigree Editor
WebElement element =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='circle' and @cy='272' and @cx='300' and @fill='#808080' and @opacity='0.3'][1]"));
Actions builder = new Actions(driver);
Thread.sleep(10000);
builder.moveToElement(element).click(element);
builder.perform();
WebElement image3 =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='rect' and @x='644' and @y='504' and @transform='matrix(1.08,0,0,1.08,-54.72,-43.52)'][1]"));
boolean clicked = false;
do{
try {
Actions builder2 = new Actions(driver);
builder2.moveToElement(image3).click(image3);
builder2.perform();
} catch (WebDriverException e) {
System.out.println("Exception " + e);
} finally {
clicked = true;
}
} while (!clicked);
// Fecthing all the text
List<WebElement>text=driver.findElements(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='text']//*[name()='tspan']"));
for(WebElement t:text){
System.out.println("Name is " + t.getText());
//if(t.getText().equals("RDPBMULTI1_FN RDPBMULTI1_SN ")){
//select it
}
WebElement image3 =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='rect' and @x='644' and @y='504' and @class='pedigree-node-shadow' and @fill='#808080'][1]"));
Actions builder2 = new Actions(driver);
builder2.moveToElement(image3).click(image3);
builder2.perform();
Thread.sleep(10000);
//WebElement UAT=driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='text'][3]/*[name()='tspan']"));
/* WebElement rdp9841=driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='text'][1]"));
WebElement rdp9833=driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='text'][4]"));
WebElement rdp9825=driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='text'][7]"));
WebElement rdp9817=driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='text'][10]"));
System.out.println("Name is " + UAT.getText());
Thread.sleep(10000);
WebElement image3 =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='rect' and @class='pedigree-node-shadow' and @x='164']"));
Actions builder2 = new Actions(driver);
builder2.moveToElement(image3).click(image3);
builder2.perform();
if(UAT.equals("UAT TEST123 UAT TEST123")){
Thread.sleep(10000);
WebElement image3 =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='rect' and @class='pedigree-node-shadow' and @x='164']"));
Actions builder2 = new Actions(driver);
builder2.moveToElement(image3).click(image3);
builder2.perform();
}
// Thread.sleep(10000);
//close popup
/* driver.findElement(By.xpath("//div[@class='menu-box']//span[@class='close-button']")).click();
WebElement element =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='circle' and @cy='272' and @cx='300' and @fill='#808080' and @opacity='0.3'][1]"));
Actions builder = new Actions(driver);
Thread.sleep(10000);
builder.moveToElement(element).click(element);
builder.perform();
//close popup
//driver.findElement(By.xpath("//div[@class='menu-box']//span[@class='close-button']")).click();
//Thread.sleep(20000);
WebElement image3 =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='rect' and @class='pedigree-node-shadow' and @x='644' and @y='504']"));
Actions builder2 = new Actions(driver);
builder2.moveToElement(image3).click(image3);
//WebElement image3 =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='rect' and @x='644' and @y='504' and @class='pedigree-node-shadow' and @fill='#808080'][1]"));
String js = "arguments[0].style.height='auto'; arguments[0].style.visibility='visible';";
// Execute the Java Script for the element which we find out
((JavascriptExecutor) driver).executeScript(js, image3);
// Click on element
image3.click();
JavascriptExecutor executor = (JavascriptExecutor) driver;
executor.executeScript("arguments[0].click();", image3);
//close popup
// driver.findElement(By.xpath("//div[@class='menu-box']//span[@class='close-button']")).click();
// Thread.sleep(10000);
WebElement element1 =driver.findElement(By.xpath("//div[@id='canvas']//*[name()='svg']//*[name()='path' and @d='M828,272L773,272']"));
Actions builder1 = new Actions(driver);
builder1.moveToElement(element1).click(element1);
*/
}
}
| 7b42397f54fefe71c32f081de86fc3d6bdffe237 | [
"Java"
] | 1 | Java | Sreekaram/RemoteACT | cd3b067b70e85c4f1d1346f5b60f35313f8694e9 | 711ec8b9a7c98a3a2a2b6a81dc41267fda5e6278 | |
refs/heads/master | <repo_name>randy-girard/MailApp<file_sep>/.env.example
IMAP_USER="<EMAIL>"
IMAP_PASSWORD="<PASSWORD>"
<file_sep>/config/routes.rb
Rails.application.routes.draw do
resources :folders, :id => /.*/ do
resources :messages
end
end
<file_sep>/app/controllers/application_controller.rb
require 'net/imap'
require 'mail'
class ApplicationController < ActionController::Base
# Prevent CSRF attacks by raising an exception.
# For APIs, you may want to use :null_session instead.
protect_from_forgery with: :exception
before_filter :open_imap
private
def open_imap
Rails.logger.info ENV.inspect
@folders = []
@messages = []
if @imap.nil?
@imap = Net::IMAP.new(ENV["IMAP_HOST"], 993, usessl = true, certs = nil, verify = false)
@imap.login(ENV["IMAP_USER"], ENV["IMAP_PASSWORD"])
begin
@folders = @imap.list('*', '*')
@folder = params[:folder_id]
if params[:id].present?
@imap.select(params[:folder_id])
msg = @imap.fetch(params[:id].to_i,'RFC822')[0].attr['RFC822']
mail = Mail.read_from_string msg
if mail
@message =
{
:id => params[:id],
:from => mail.from,
:subject => mail.subject,
:date => mail.date,
:text => mail.text_part.try(:body),
:html => mail.html_part.try(:body),
:raw => mail
}
else
@message = {}
end
elsif params[:folder_id].present?
@imap.select(params[:folder_id])
@messages = @imap.search(["ALL"]).map do |message_id|
msg = @imap.fetch(message_id,'RFC822')[0].attr['RFC822']
mail = Mail.read_from_string msg
if mail
{
:id => message_id,
:from => mail.from,
:subject => mail.subject,
:date => mail.date,
:text => mail.text_part.try(:body),
:html => mail.html_part.try(:body),
:raw => mail
}
else
{}
end
end
end
ensure
@imap.logout
@imap.disconnect
end
end
end
end
| bc2613ea57d2271b679d6b369e1d5c1b83b6a501 | [
"Ruby",
"Shell"
] | 3 | Shell | randy-girard/MailApp | 24150bd0aab6bc1abf56a26435d59f04c507928f | a0e5a5f69c28ddba6a4973ad8dc81b9bdb36c6db | |
refs/heads/master | <repo_name>uniquerockrz/BackupHelper<file_sep>/Readme.md
### THIS APP IS NO LONGER MAINTAINED
Bug reports, feature and pull requests will no longer be entertained.
This is a simple program I wrote using C because I was too lazy to see individual folders to take my backup. Most backup program allow you to sync your hard disk with external rewritable drive, such as pen drives, external hard drive or a upload server. This one will help you to sync your drive (or a part of it) with non-rewriteable media such as DVDs which are cheapest for taking backups.
Using this program, you can:
* Check what files you need to add in your backup drive
* Check what files have been modified since the last backup
* Know if two directory structure are synced or not.
* Check what files have been deleted since the last backup
The program works with the help of two input files, an old filelist text file and a new file list text file. This would work in Linux systems (I have tested it individually), sorry I dont know about windows as i dont have that installed. 8)
Copy and save the program as BackupHelper.c, compile the program using gcc using the following code
```bash
$ gcc -Wall BackupHelper.c -o BackupHelper
```
Now, first take a backup of your hard disk (or a folder in it, say music) in a DVD. Now cd to that folder and create a filelist of that folder and save it in any name (say a.txt)
```bash
$ cd music
$ tree -fis > a.txt
```
Copy this file in the folder the program executable is present.
Note: If you dont use the -s option, the program wont check for file modifications or changes, it will only check for content added.
Now after some days (maybe when you have added more content in your music folder) cd to the folder you want to take the backup. Again create a filelist of that folder using the following commands.
```bash
$ cd music
$ tree -fis > b.txt
```
Copy the b.txt to the program's executable folder. Now you need to compare the both the files to see the changes in music folder. For that, type ./BackupHelper oldfilelist.txt newfilelist.txt and press enter.
```bash
$ ./BackupHelper a.txt b.txt
```
The program would display all the files and folders that have been added after your last backup. Now you can burn the changed or added files in your backup dvd.


Delete the old filelist file. Keep the new. Next time you want to take backup, cd to the folder and create the filelist file using tree -fis >filelist.txt command. Compare the older file with new one and you are done.
Please note: This program doesnt takes your backups, but rather helps you to, so the name is BackupHelper. For encryption or more advanced options, you can use deja dup. However you have to write another DVD everytime you backup, thus increasing file-redundancy and costs. This will help you keeping the directories synced, the backup, however, you have to do manually.
If no changes have been made since the last backup, the program would display a message saying so. It also displays the files that were present earlier but have been recently deleted. You can also use it to know if two folders are synced.
<file_sep>/BackupHelper.c
#include <stdio.h>
char oldfile[10000][200], newfile[10000][200]; // stores the file content
int nold, nnew; // number of files in each file list
int main(int argc, char *argv[]){
int copytoarray(char filename[100], char arr[10000][200]);
void comparefiles();
// copying the file content in an array
nold=copytoarray(argv[1], oldfile);
nnew=copytoarray(argv[2], newfile);
printf("\n");
// comparing the files and displaying the differences
comparefiles(newfile, oldfile);
return 0;
}
int copytoarray(char filename[100], char arr[10000][200]){
FILE *fp;
int i,n;
fp=fopen(filename,"r");
i=0;
while(fgets(arr[i],200,fp)!=NULL)
i++;
n=i;
return n;
}
void comparefiles(){
int i,j,n=0;
int comparestr(char str1[200], char str2[200]);
for(i=0,j=0;i<(nnew-1);){
if(j>(nold-1)){
n++;
printf("%d Changed/Added %s\n",n, newfile[i]);
i++;
j=0;
}
if(comparestr(newfile[i], oldfile[j])){
i++;
oldfile[j][0]='\0';
j=0;
}
else j++;
}
if(n==0)
printf("Backup is up to date! No notable difference found.\n");
for(i=0;i<(nold-2);i++){
if(oldfile[i][0]!='\0'){
n++;
printf("%d Deleted : %s\n", n, oldfile[i]);
}
}
}
int comparestr(char str1[200],char str2[200]){
int i;
if(str2[0]=='\0'){
return 0;
}
else{
for(i=0;str1[i]!='\n';i++){
if(str1[i]!=str2[i])
return 0;
}
}
return 1;
}
| 36ff5f947bf1fb6fe9ac07084193057ab45b7026 | [
"Markdown",
"C"
] | 2 | Markdown | uniquerockrz/BackupHelper | cf0843a451b268832923738ebff03d14440b9f3a | 6a4a60e78d78e789c43c9e6f468d0c39bf5d5ac0 | |
refs/heads/master | <file_sep># Script install Cadson Demak Github Fonts
script inspired from [Web-Font-Load](https://github.com/qrpike/Web-Font-Load)<file_sep>#!/bin/bash
# OS detection
osdetect=$(uname)
file_path="unknown"
if [[ "$osdetect" == 'Darwin' ]]; then
file_path="/Library/Fonts/"
elif [[ "$osdetect" == 'Linux' ]]; then
if [[ -d /usr/local/share/fonts/ ]]; then # Debian/Ubuntu and others.
file_path="/usr/local/share/fonts/"
elif [[ -d /usr/share/fonts/ ]]; then # OpenSUSE, Arch and other distros using this directory structure
sudo mkdir -p /usr/share/fonts/google/
file_path="/usr/share/fonts/google/"
else # Fallback to installing fonts locally to the user, this is a safe bet, as several distros use this location.
mkdir -p ~/.fonts
file_path="~/.fonts/"
fi
fi
clear
echo "Installing all <NAME>'s (Google 13+1 Thai Fonts) onto your System"
echo "Downloading the fonts..."
mkdir -p tmp/download tmp/fonts
curl -q -o "./tmp/download/#1.zip" -LO https://github.com/cadsondemak/\{Charm,Bai-Jamjuree,Niramit,Srisakdi,Fah-Kwang,Chakra-Petch,Thasadith,Sarabun,Krub,Kodchasan,K2D,Koho,Mali,Charmonman\}/archive/master.zip
echo "Extracting the fonts..."
find tmp/download -name '*.zip' -exec sh -c 'unzip -q -d `dirname {}` {} "*.ttf"' ';'
#find tmp/download -type f -name "*.ttf" -exec cp {} tmp/fonts \;
# Install to system
sudo find tmp/download -type f -name "*.ttf" -exec cp {} $file_path \;
# echo "Fonts installed; Cleaning up files..."
echo "Cleaning up files..."
rm -rf tmp/download
rm -rf tmp/fonts
echo "All done! All Google 13+1 Thai Fonts installed." | d6a244070df6ead2c92101ce046c1b702f193bc8 | [
"Markdown",
"Shell"
] | 2 | Markdown | dahoba/google-thai-fonts-dl-script | 25880c010ce1462435e3ebf2066c3236f8a72b95 | b6ea19306d26735111f24636b935a0aed18c5a81 | |
refs/heads/master | <repo_name>jcarlosj/Learning-Javascript-Project-Simon-Dice<file_sep>/js/simon-dice.js
/* <NAME>: */
const levels = 15;
let keys = generateKeys( levels );
// Siguiente nivel
function nextLevel( currentLevel ) {
console .log( 'Debe mostrar ', currentLevel + 1, ' teclas' );
// Valida si se ha superado el
if( currentLevel == levels ) {
return swal({ // return detiene la ejecución
timer: 1000,
title: 'Wow! Excelente has ganado!',
type: 'success'
});
}
swal({ // Muestra el nivel actual
timer: 1000,
title: `Nivel ${ currentLevel + 1 }`,
showConfirmButton: false
});
/* Juego: Muestra la secuencia de teclas por nivel al jugador */
for( let i = 0; i <= currentLevel; i++ ) {
setTimeout( () => activateEl( keys[ i ] ), 1000 * ( i + 1 ) + 1000 );
}
/* Juego: espera que el jugador presione la primera tecla (TURNO) */
let ronda = 0,
currentKey = keys[ ronda ];
// Agrega manejador de eventos
window .addEventListener( 'keydown', onkeydown );
// Internal Function: Valida cuando una tecla es presionada
function onkeydown( event ) {
// Valida si la tecla presionada es igual a la actual en la secuencia generada
if( event .keyCode == currentKey ) {
activateEl( currentKey, { success: true } ); // Activa el estilo 'success' por que acerto
ronda++; // Avanza a la siguiente ronda
if( ronda > currentLevel ) { // Valida el que la ronda sea mayor que el nivel (en la secuencia)
window .removeEventListener( 'keydown', onkeydown ); // Elimina el manejador de eventos
setTimeout( () => {
nextLevel( ronda ); // Avanza al siguiente nivel
}, 1500 );
}
currentKey = keys[ ronda ]; // Reasigna la tecla actual de la secuencia de teclas generadas (la siguente en la secuencia)
}
else {
activateEl( event .keyCode, { fail: true } ); // Activa el estilo 'fail' por que falló
window .removeEventListener( 'keydown', onkeydown ); // Elimina el manejador de eventos
setTimeout( () => {
swal({
timer: 3000,
title: 'Lo siento, perdiste. Vuelve a intentarlo!',
text: 'Quiéres jugar de nuevo?',
showCancelButton: true,
confirmButtonText: 'Sí',
cancelButtonText: 'No',
confirmButtonColor: '#3085d6',
cancelButtonColor: '#d33',
closeOnConfirm: true
}).then( result => {
if ( result .value ) {
keys = generateKeys( levels );
nextLevel( 0 );
}
});
}, 2000 );
}
}
}
/* Pruebas */
nextLevel( 0 ); // Nivel 1 (El inicio del juego)
//nextLevel( 6 ); // Nivel 7
//nextLevel( 15 ); // Nivel 16 (no existe, has ganado)
// Genera una secuencia de keyCodes aleatorios de teclas 'a' a la 'z'(65 a 90)
function generateKeys( levels ) {
// Retorna un Array con los valores aleatorios en cada una de las posiciones definidas ( levels )
return new Array( levels ) .fill( 0 ) .map( generateRandomKey );
/* NOTA:
- new Array( levels ): Instancia un Objeto de tipo 'Array' con una cantidad de posiciones definidas.
- fill( 0 ): llena el Array con CEROS, eso se hace por que no se puede mapear un array vacío.
- map( generateRandomKey ): 'Callback' con el que se mapean cada uno de los campos y se le asigna el valor retornado por el mismo.
*/
}
// Genera aleatoriamente un número entre 65 y 90
function generateRandomKey() {
const minKeyCode = 65, // a
maxKeyCode = 90; // z
return Math .round( Math .random() * ( maxKeyCode - minKeyCode ) + minKeyCode );
}
// Obtiene el elemento HTML que tenga el keyCode respectivo
function getElementByKeyCode( keyCode ) {
return document .querySelector( `[data-key="${keyCode}"]` );
}
// Activa estilo al presionar tecla de acuerdo al estado
function activateEl( keyCode, options = {} ) {
const el = getElementByKeyCode( keyCode );
el .classList .add( 'active' ); // Agrega una clase al listado de clases del elemento
if( options .success ) {
el .classList .add( 'success' ); // Agrega una clase al listado de clases del elemento
}
else if( options .fail ) {
el .classList .add( 'fail' ); // Agrega una clase al listado de clases del elemento
}
// Temporizador para eliminar clases agregadas al elemento
setTimeout( () => deactivateEl( el ), 1000 );
}
// Desactiva estilo al presionar tecla de acuerdo al estado
function deactivateEl( el ) {
el .className = 'key'; // Elimina el listado de clases y define exclusivamente la clase 'key'
}
| b98ec3c2038af3547faac6133572fae63cff04c5 | [
"JavaScript"
] | 1 | JavaScript | jcarlosj/Learning-Javascript-Project-Simon-Dice | c6998cff7d760a52151925507c4a2505a1abe34a | be93cf17f59d2e3bb53cbd9341eb3c15a38034e4 | |
refs/heads/master | <file_sep>#!/bin/sh /etc/rc.common
#
#Start and Stop functions to run the ipkg package
#
PIDFILE_0=/var/run/wifi_dump_0.pid
PIDFILE_1=/var/run/wifi_dump_1.pid
HASH_KEY=/etc/bismark/passive.key
START=99
start(){
if [ ! -f $HASH_KEY ]; then
dd if=/dev/urandom of=$HASH_KEY bs=1 count=16 2>/dev/null
echo "Generated fresh anonymization key"
fi
mkdir -p /tmp/bismark-uploads/mac-analyzer
mkdir -p /tmp/mac-analyzer
iw phy phy0 interface add phe0 type monitor flags fcsfail control otherbss 2>/dev/null
p0=$?
if [ "$p0" -eq "0" ];then
mon0='phe0'
echo "phe0 created "
else
echo "Err: can't creat phe0"
exit 1
fi
ifconfig $mon0 up
p0=$?
if [ "$p0" -eq "0" ];then
echo "radio 0 interface up"
else
echo "Err: can't turn radio 0 interface up"
exit 1
fi
iw phy phy1 interface add phe1 type monitor flags fcsfail control otherbss 2>/dev/null
p1=$?
if [ "$p1" -eq "0" ];then
mon1='phe1'
echo "phe1 created "
else
echo "Err: can't creat phe1"
exit 1
fi
ifconfig $mon1 up
p1=$?
if [ "$p1" -eq "0" ];then
echo "radio 0 interface up"
else
echo "Err: can't turn radio 0 interface up"
exit 1
fi
#check the argument
if [ -f $PIDFILE_0 ]; then
echo "pidfile $PIDFILE_0 already exists; mac analyzer already running"
exit 1
fi
if [ -f $PIDFILE_1 ]; then
echo "pidfile $PIDFILE_1 already exists; mac analyzer already running"
exit 1
fi
start-stop-daemon -S \
-x /tmp/usr/bin/wifi_dump \
-p $PIDFILE_0 \
-m -b $mon0
echo "installed on phe0 interface; now running phe1 interface"
sleep 3
start-stop-daemon -S \
-x /tmp/usr/bin/wifi_dump \
-p $PIDFILE_1 \
-m -b $mon1
}
stop(){
[ -f $PIDFILE_0 ] && {
start-stop-daemon -K -q -p $PIDFILE_0 -s TERM
rm -f $PIDFILE_0
}
[ -f $PIDFILE_1 ] && {
start-stop-daemon -K -q -p $PIDFILE_1 -s TERM
rm -f $PIDFILE_1
}
# ifconfig phe0 down
# p1=$?
# if [ "$p1" -eq "0" ];then
# echo "phe0 turned down"
# fi
# ifconfig phe1 down
# p1=$?
# if [ "$p1" -eq "0" ];then
# echo "phe1 turned down"
# fi
iw dev phe0 del 2>/dev/null
p1=$?
if [ "$p1" -eq "0" ];then
echo "deleted phe0 interface "
fi
iw dev phe1 del 2>/dev/null
p1=$?
if [ "$p1" -eq "0" ];then
echo "deleted phe1 interface "
fi
}
restart(){
stop
start
}
<file_sep># Copyright (C) 2006-2011 OpenWrt.org
#
# This is free software, licensed under the GNU General Public License v2.
# See /LICENSE for more information.
#
include $(TOPDIR)/rules.mk
PKG_NAME:=wifi-dump-tmpfs
PKG_RELEASE:=12
PKG_VERSION:=HEAD
PKG_SOURCE:=$(PKG_NAME)-$(PKG_VERSION).tar.gz
PKG_SOURCE_URL:=git://github.com/abnarain/wifi_dump-tmpfs.git
PKG_SOURCE_VERSION:=$(PKG_VERSION)
PKG_SOURCE_SUBDIR:=$(PKG_NAME)-$(PKG_VERSION)
PKG_SOURCE_PROTO:=git
#GIT_URL:=git:/
#GIT_URL:=src/
include $(INCLUDE_DIR)/package.mk
define Package/wifi-dump-tmpfs
SECTION:=net
CATEGORY:=Network
TITLE:=BISmark Wireless Measurements tool
URL:=http://doesnotexist.com
DEPENDS:=+zlib +bismark-data-transmit
endef
define Package/bismark-active-tmpfs/description
This tool collects wireless traces in monitor mode. This tool is a part of infrastructure to understand wireless home networks.
endef
define Package/wifi-dump-tmpfs/install
$(INSTALL_DIR) $(1)/usr/bin
$(INSTALL_DIR) $(1)/etc/init.d
$(INSTALL_BIN) $(PKG_BUILD_DIR)/wifi_dump $(1)/usr/bin/wifi_dump.bin
$(INSTALL_BIN) ./files/etc/init.d/wifi_dump $(1)/etc/init.d/wifi_dump
$(INSTALL_BIN) ./files/usr/bin/wifi_dump $(1)/usr/bin/wifi_dump
endef
define Package/wifi-dump-tmpfs/postinst
#!/bin/sh
#killall wifi_dump
#rm /var/run/wifi_dump.pid
start-stop-daemon -K -q -p /var/run/wifi_dump.pid -s TERM
/tmp/etc/init.d/wifi_dump restart
endef
define Package/wifi-dump-tmpfs/prerm
#!/bin/sh
/tmp/etc/init.d/wifi_dump stop
endef
$(eval $(call BuildPackage,wifi-dump-tmpfs))
| 868bef4e239884ba4ef5e71c03047ba88bdbc571 | [
"Makefile",
"Shell"
] | 2 | Shell | abnarain/bismark-packages | 68fc2ba373fcccb26d40fb33d0b32b793e270382 | b432fbc9bd177803e9de9a59b8c17f6f3af0def2 | |
refs/heads/master | <file_sep><?php
namespace Pythagus\Lydia\Contracts;
/**
* Interface LydiaState
* @package Pythagus\Lydia\Contracts
* @see https://lydia-app.com/doc/api/#api-Payment-State
*
* @author: <NAME>
*/
interface LydiaState {
/**
* Unknown transaction state.
*
* @const int
*/
public const UNKNOWN = -1 ;
/**
* State of a waiting payment. A transaction
* request was addressed to Lydia.
*
* @const int
*/
public const WAITING_PAYMENT = 0 ;
/**
* The payment was confirmed by Lydia.
*
* @const int
*/
public const PAYMENT_CONFIRMED = 1 ;
/**
* The payment was refused by the bank.
*
* @const int
*/
public const REFUSED_BY_PAYER = 5 ;
/**
* The payment was cancelled by the client
* before the end.
*
* @const int
*/
public const CANCELLED_BY_OWNER = 6 ;
}<file_sep><?php
namespace Pythagus\Lydia\Traits;
use Pythagus\Lydia\Lydia;
/**
* Trait HasLydiaProperties
* @package Pythagus\Lydia\Traits
*
* @author: <NAME>
*/
trait HasLydiaProperties {
/**
* Determine whether the website is in a
* lydia debugging mode.
*
* @return bool
*/
protected function isTestingMode() {
return boolval($this->getConfig('debug', false)) ;
}
/**
* Get the PaymentLydia server's URL
*
* @return string
*/
protected function getLydiaURL() {
return $this->getLydiaCredential('url_server') ;
}
/**
* Get the PaymentLydia vendor token.
*
* @return string
*/
protected function getLydiaVendorToken() {
return $this->getLydiaCredential('vendor_token') ;
}
/**
* Get the PaymentLydia private token.
*
* @return string
*/
protected function getLydiaPrivateToken() {
return $this->getLydiaCredential('private_token') ;
}
/**
* Get the property in the lydia.php file
* using the debug value.
*
* @param string $property
* @return string
*/
protected function getLydiaCredential(string $property) {
$mode = $this->isTestingMode() ? 'debug' : 'prod' ;
return $this->getConfig('credentials.'.$mode.'.'.$property) ;
}
/**
* Get the config value.
*
* @param string $key
* @param null $default
* @return mixed|null
*/
protected function getConfig(string $key, $default = null) {
return Lydia::getInstance()->config($key, $default) ;
}
}<file_sep><?php
namespace Pythagus\Lydia\Http;
use Pythagus\Lydia\Contracts\LydiaException;
/**
* Class RefundRequest
* @package Pythagus\Lydia\Http
*
* @property string identifier
*
* @author: <NAME>
*/
class RefundRequest extends LydiaRequest {
/**
* Payment identifier given in the
* Lydia return after a payment.
*
* @var string
*/
private $identifier ;
/**
* @param string $identifier
*/
public function __construct(string $identifier) {
$this->identifier = $identifier ;
}
/**
* Execute the request.
*
* @return mixed
* @throws LydiaException
*/
protected function run() {
$this->setRequestParameters() ;
$result = $this->requestServer('refund') ;
/**
* This method prevents to the Lydia problems that
* can occurred. The incoming exception should not
* be caught here to be printed to the client.
*
* @throws LydiaException
*/
$this->lydiaResponseContains($result, 'error', true) ;
/*
* Here, the response should be a Std object
* with keys:
* - error: Set to 0 if there is no error.
*/
return $result['error'] ;
}
/**
* Get the list of required parameters for
* the request.
*
* @return array
*/
protected function shouldHaveParameters() {
return ['amount'] ;
}
/**
* Set the default parameters.
*
* @return void
*/
protected function setRequestParameters() {
$amount = $this->parameters['amount'] ;
$this->clearParameters() ;
$this->setParameter('transaction_identifier', $this->identifier) ;
$this->setParameter('amount', $amount) ;
$this->setParameter('signature', $this->generateSignature($this->parameters)) ;
$this->setParameter('vendor_token', $this->getLydiaVendorToken()) ;
}
}<file_sep><?php
namespace Pythagus\Lydia\Traits;
/**
* Trait HasParameters
* @package Pythagus\Lydia\Helpers
*
* @property array parameters
*
* @author: <NAME>
*/
trait HasParameters {
/**
* Request parameters.
*
* @var array
*/
protected $parameters = [] ;
/**
* Set the parameter to the given value.
*
* @param string $field
* @param $value
*/
public function setParameter(string $field, $value) {
$this->parameters[$field] = $value ;
}
/**
* Reset all the parameters.
*
* @return void
*/
public function clearParameters() {
$this->parameters = [] ;
}
/**
* Get the list of required parameters for
* the request.
*
* @return array
*/
protected function shouldHaveParameters() {
return [] ;
}
/**
* Set the default parameters.
*
* @return void
*/
protected function setDefaultParameters() {
//
}
}<file_sep><?php
namespace Pythagus\Lydia\Http;
use Pythagus\Lydia\Contracts\LydiaException;
/**
* Class PaymentStateRequest
* @package Pythagus\Lydia\Http
*
* @property string requestUUID
*
* @author: <NAME>
*/
class PaymentStateRequest extends LydiaRequest {
/**
* Request UUID to check.
* This attribute was given in the PaymentRequest response.
*
* @var string
*/
private $requestUUID ;
/**
* @param string $requestUUID
*/
public function __construct(string $requestUUID) {
$this->requestUUID = $requestUUID ;
}
/**
* Execute the request.
*
* @return mixed
* @throws LydiaException
*/
protected function run() {
$result = $this->requestServer('state') ;
/**
* This method prevents to the Lydia problems that
* can occurred. The incoming exception should not
* be caught here to be printed to the client.
*
* @throws LydiaException
*/
$this->lydiaResponseContains($result, 'error', false) ;
$this->lydiaResponseContains($result, 'state', true) ;
/*
* Here, the response should be a Std object
* with keys:
* - state: Status of the remote request. See LydiaState contract interface.
* - used_ease_of_payment: Specify if the user used ease of payment or not.
*/
return intval($result['state']) ;
}
/**
* Set the default parameters.
*
* @return void
*/
protected function setDefaultParameters() {
// Vendor token of the business that done the request.
$this->setParameter('vendor_token', $this->getLydiaVendorToken()) ;
// UUID of the remote payment request.
$this->setParameter('request_uuid', $this->requestUUID) ;
}
}<file_sep><?php
namespace Pythagus\Lydia\Contracts;
use Exception;
use Throwable;
/**
* Class LydiaException
* @package Pythagus\Lydia\Contracts
*
* @author: <NAME>
*/
class LydiaException extends Exception {
/**
* @param string $message
* @param int $code
* @param Throwable|null $previous
*/
public function __construct($message = "", $code = 0, Throwable $previous = null) {
parent::__construct(
"Lydia : " . $message, $code, $previous
) ;
}
}<file_sep># Lydia payment
[Lydia](https://lydia-app.com/fr) is an online-payment solution. This package presents an implementation of this tool in
an Object-Oriented view. This is a stand-alone package.
## Version
This package works since PHP 7.3. Please refer to the next table to check whether your PHP version is compatible with this package.
|Package version|PHP version|
|---------------|-----------|
| 1.x | 7.x, 8.x |
## Installation
### With composer
Use the package manager [composer](https://getcomposer.org/) to install this package.
```bash
composer require pythagus/lydia
```
### Without package manager
You can clone the current repository and completely use this package. You need to make (or use) an autoload package
(like [composer](https://getcomposer.org/)) to correctly discover the files.
## Usage
This section describes how to correctly and fully use this package.
### The Lydia facade
The ```Pythagus\Lydia\Lydia``` class allows you to custom the Lydia main interactions with your application.
**You need to extend this class**. The package won't work otherwise. You can refer to the [example/MyLydiaFacade](example/MyLydiaFacade)
class to have an example how to override this class.
When you override the ```Lydia``` class, you need to àdd the next line to be taking into account:
```php
Lydia::setInstance(new MyLydiaFacade()) ;
```
If you are using a framework, you can add this line when your application is starting. If you are not, you need to add this
line before every request you make.
**Note :** if you are using a framework, you will probably have a redirect response manager. So you can override the ```Lydia.redirect()```
method to use your framework instead.
### Configuration
You have an example of a configuration file in the [example folder](example). The ```MyLydiaFacade``` class show you what you should
override to adapt this package to your application. I suggest you copying the ```lydia.php``` config file and only add your personal keys.
#### Properties
The ```properties``` key in the ```example/lydia.php``` config file allows you to declare some properties that won't change from a
request to another (like the currency).
### Lydia requests
For now, the package implements 3 Lydia's requests:
#### PaymentRequest
Make a payment request to the Lydia's server. You need to use the ```setFinishCallback()``` method to define the return route using by
Lydia to redirect the user. You also need to define **amount** and **recipient** parameters like:
```php
$request = new PaymentRequest() ;
$request->setFinishCallback('my/route') ;
$data = $request->execute([
'amount' => 5,
'recipient' => '<EMAIL>'
]) ;
// Save your data.
return $request->redirect() ;
```
The ```execute()``` method will redirect the user to the Lydia's page. After payment, the user will be redirected to ```my/route```.
#### PaymentStateRequest
Check the state of a payment. You need to set the ```request_uuid``` that is given in the ```Lydia.savePaymentData()``` array when the payment is requested.
You can execute the request like:
```php
$request = new PaymentStateRequest("payment_request_uuid") ;
$state = $request->execute() ;
```
#### RefundRequest
Refund partially or globally a transaction. You need to set the ```transaction_identifier``` that is given in the ```PaymentRequest``` finish route.
You need to add the **amount** parameter.
You can execute the request like:
```php
$request = new RefundRequest("payment_transaction_identifier") ;
$status = $request->execute([
'amount' => 10
]) ;
```
## Contributing
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.
## License
[MIT](LICENSE)<file_sep><?php
namespace Pythagus\Lydia\Http;
use anlutro\cURL\cURL;
use Pythagus\Lydia\Lydia;
use Pythagus\Lydia\Traits\HasParameters;
use Pythagus\Lydia\Contracts\LydiaException;
use Pythagus\Lydia\Traits\HasLydiaProperties;
use Pythagus\Lydia\Exceptions\InvalidLydiaResponseException;
/**
* Class LydiaRequest
* @package Pythagus\Lydia\Http
*
* @author: <NAME>
*/
abstract class LydiaRequest {
use HasParameters, HasLydiaProperties ;
/**
* Execute the request.
*
* @return mixed
*/
abstract protected function run() ;
/**
* Get a Lydia instance.
*
* @return Lydia
*/
protected function lydia() {
return Lydia::getInstance() ;
}
/**
* Execute the request adding some parameters.
*
* @param array $parameters
* @return mixed
* @throws LydiaException
*/
public function execute(array $parameters = []) {
$this->setDefaultParameters();
$this->addPropertiesOnArray($this->getConfig('properties', [])) ;
$this->addPropertiesOnArray($parameters) ;
foreach($this->shouldHaveParameters() as $parameter) {
if(! array_key_exists($parameter, $this->parameters)) {
throw new LydiaException(class_basename($this) . " should have a parameter : $parameter") ;
}
}
return $this->run() ;
}
/**
* Set the properties listed in the given array.
*
* @param array $properties
*/
protected function addPropertiesOnArray(array $properties) {
foreach($properties as $key => $value) {
$this->setParameter($key, $value) ;
}
}
/**
* Check whether the Lydia response has the
* expected format.
*
* @param array $response
* @param $keys
* @param bool $shouldHave
* @throws LydiaException
*/
protected function lydiaResponseContains(array $response, $keys, bool $shouldHave) {
if(! is_array($keys)) {
$keys = [$keys] ;
}
/*
* We test every keys contained in
* the $keys variable.
*/
foreach($keys as $key) {
$has = isset($response[$key]) ;
/*
* There is an error if:
* -> The response should have the $key field and has not one.
* -> The response should not have the $key field and has one.
*/
if($shouldHave xor $has) {
throw new InvalidLydiaResponseException(
"Lydia's response " . ($shouldHave ? "should" : "shouldn't") . " have $key attribute", $response
) ;
}
}
}
/**
* Generate the request signature.
*
* @param array $param
* @return string
* @see: https://lydia-app.com/doc/api/#signature
*/
protected function generateSignature(array $param) {
/*
* We are making a copy of the
* given param to leave the given
* value intact.
*/
$data = $param ;
// Alphabetical ordering
ksort($data) ;
return md5(
http_build_query($data) . '&' . $this->getLydiaPrivateToken()
) ;
}
/**
* Make a request server call.
*
* @param string $route : config key (lydia.url.$key) in lydia.php config file.
* @return array
*/
protected function requestServer(string $route) {
$response = (new cURL())->post(
$this->getLydiaURL() . $this->getConfig('url.' . $route), $this->parameters
) ;
return json_decode($response->getBody(), true) ;
}
}<file_sep><?php
use Pythagus\Lydia\Lydia;
/**
* Class MyLydiaFacade
*
* @author: <NAME>
*/
class MyLydiaFacade extends Lydia {
/**
* Get the Lydia's configuration.
*
* @return array
*/
protected function setConfigArray() {
return require __DIR__ . '/lydia.php' ;
}
/**
* Format the callback URL to be valid
* regarding the Lydia server.
*
* @param string $url
* @return string
*/
public function formatCallbackUrl(string $url) {
return 'https://www.google.com/' . $url ;
}
}<file_sep><?php
namespace Pythagus\Lydia\Exceptions;
use Pythagus\Lydia\Contracts\LydiaException;
/**
* Class InvalidLydiaResponseException
* @package Pythagus\Lydia\Exceptions
*
* @property array response
*
* @author: <NAME>
*/
class InvalidLydiaResponseException extends LydiaException {
/**
* Make a new InvalidLydiaResponse exception.
*
* @param string $message : original exception message.
* @param array $response
*/
public function __construct(string $message, array $response) {
parent::__construct(
$message . '(response : ' . json_encode($response) . ')'
) ;
}
}<file_sep><?php
namespace Pythagus\Lydia\Contracts;
/**
* These error codes were generated from logs after
* Lydia usages. This is not an exhaustive list.
*
* Class PaymentErrorCode
* @package Pythagus\Lydia\Contracts
*
* @author: <NAME>
*/
interface PaymentErrorCode {
/**
* Invalid email address given (recipient).
*
* @var integer
*/
public const INVALID_RECIPIENT = 3 ;
/**
* The recipient cannot receive more payment requests.
*
* @var integer
*/
public const FLOODED_RECIPIENT = 4 ;
/**
* The recipient was blocked.
*
* @var integer
*/
public const BLOCKED_RECIPIENT = 6 ;
}<file_sep><?php
namespace Pythagus\Lydia\Traits;
use Throwable;
/**
* Trait LydiaTools
* @package Pythagus\Lydia\Traits
*
* @author: <NAME>
*/
trait LydiaTools {
/**
* We are trying to take the transaction_identifier
* token in the GET Lydia's response.
*
* @return string|null
*/
public static function getTransactionIdentifier() {
try {
return $_GET['transaction'] ?? null ;
} catch(Throwable $ignored) {
return null ;
}
}
}<file_sep><?php
namespace Pythagus\Lydia\Traits;
use Pythagus\Lydia\Contracts\LydiaException;
/**
* Trait HasConfig
* @package Pythagus\Lydia\Traits
*
* @property array configs
*
* @author: <NAME>
*/
trait HasConfig {
/**
* Lydia's configurations
*
* @var array
*/
private $configs = [] ;
/**
* Get the config value identified by
* the given key.
*
* @param string $key
* @param null $default
* @return mixed|null
*/
public function config(string $key, $default = null) {
$array = $this->configs ;
if(count($array) <= 0) {
throw new LydiaException("No configs are loaded") ;
}
if(array_key_exists($key, $array)) {
return $array[$key] ;
}
if(strpos($key, '.') === false) {
return $array[$key] ?? $default ;
}
foreach(explode('.', $key) as $segment) {
if(array_key_exists($segment, $array)) {
$array = $array[$segment] ;
} else {
return $default ;
}
}
return $array ;
}
}<file_sep><?php
namespace Pythagus\Lydia\Exceptions;
use Pythagus\Lydia\Contracts\LydiaException;
/**
* Class LydiaErrorResponseException
* @package Pythagus\Lydia\Exceptions
*
* @property int code
* @property string message
*
* @author: <NAME>
*/
class LydiaErrorResponseException extends LydiaException {
/**
* Lydia error code.
*
* @var integer
*/
public $code ;
/**
* The returned message.
*
* @var string
*/
public $message ;
/**
* Make a new error exception.
*
* @param string $message : original exception message.
* @param array $response
*/
public function __construct(int $code, string $message) {
$this->code = $code ;
$this->message = $message ;
parent::__construct("$message (code $code)") ;
}
}<file_sep><?php
namespace Pythagus\Lydia;
use Exception;
use Pythagus\Lydia\Traits\HasConfig;
use Pythagus\Lydia\Contracts\LydiaState;
/**
* Class Lydia
* @package Pythagus\Lydia
*
* @author: <NAME>
*/
class Lydia implements LydiaState {
use HasConfig ;
/**
* The current globally available container (if any).
*
* @var Lydia
*/
protected static $instance ;
public function __construct() {
$this->configs = $this->setConfigArray() ;
}
/**
* Set the Lydia instance.
*
* @param Lydia $lydia
*/
public static function setInstance(Lydia $lydia) {
Lydia::$instance = $lydia ;
}
/**
* Get the globally available instance of the Lydia
* facade.
*
* @return static
*/
public static function getInstance() {
if(empty(Lydia::$instance)) {
Lydia::$instance = new static ;
}
return Lydia::$instance ;
}
/**
* Get the Lydia's configuration.
*
* @return array
*/
protected function setConfigArray() {
return [] ;
}
/**
* Redirect the user to the given route.
*
* @param string $route
* @return mixed
*/
public function redirect(string $route) {
header('Location: '.$route) ;
exit() ;
}
/**
* Format the callback URL to be valid
* regarding the Lydia server.
*
* @param string $url
* @return string
*/
public function formatCallbackUrl(string $url) {
throw new Exception("Should override this method") ;
}
}<file_sep><?php
return [
/*
|--------------------------------------------------------------------------
| Lydia main properties
|--------------------------------------------------------------------------
|
| This array lists the properties that won't change
| from a request to another. This properties are added
| in the LydiaRequest.executeRequest() method.
*/
'properties' => [
// Currency: only EUR and GBP are supported.
'currency' => 'EUR',
],
/*
|--------------------------------------------------------------------------
| Lydia debugging mode
|--------------------------------------------------------------------------
|
| This value determines whether the website is in a debugging mode.
| The transactions won't be move money.
*/
'debug' => true,
/*
|--------------------------------------------------------------------------
| Lydia credentials
|--------------------------------------------------------------------------
|
| This array contains the different PaymentLydia's credentials according
| to the previous debug value.
*/
'credentials' => [
/*
* Debugging mode.
*/
'debug' => [
'url_server' => 'https://homologation.lydia-app.com',
'vendor_token' => "<PASSWORD>",
'private_token' => "<PASSWORD>",
],
/*
* Production mode.
*/
'prod' => [
'url_server' => 'https://lydia-app.com',
'vendor_token' => "<PASSWORD>",
'private_token' => "<PASSWORD>",
],
],
/*
|--------------------------------------------------------------------------
| Lydia's URL
|--------------------------------------------------------------------------
|
| This array contains the available and used Lydia's url
*/
'url' => [
/*
* URL used to make transactions.
*/
'do' => '/api/request/do.json',
/*
* URL used to check the payment state.
*/
'state' => '/api/request/state.json',
/*
* URL used to refund transaction. This
* route is sometimes comment to be unusable.
*/
'refund' => '/api/transaction/refund.json',
],
] ;
<file_sep><?php
namespace Pythagus\Lydia\Http;
use Pythagus\Lydia\Lydia;
use Pythagus\Lydia\Contracts\LydiaException;
use Pythagus\Lydia\Exceptions\LydiaErrorResponseException;
/**
* Class PaymentRequest
* @package Pythagus\Lydia\Http
*
* @property string url
*
* @author: <NAME>
*/
class PaymentRequest extends LydiaRequest {
/**
* Redirection url.
*
* @var string
*/
protected $url ;
/**
* Execute the request.
*
* @return mixed
* @throws LydiaException
*/
protected function run() {
$result = $this->requestServer('do') ;
// If there is an error, then throw an exception.
if($result['error'] != 0) {
throw new LydiaErrorResponseException(
intval($result['error']), $result['message']
) ;
}
/**
* This method prevents to the Lydia problems that
* can occurred. The incoming exception should not
* be caught here to be printed to the client.
*
* @throws LydiaException
*/
$this->lydiaResponseContains($result, [
'error', 'mobile_url', 'request_uuid', 'request_id'
], true) ;
/*
* Here, the response should be a Std object
* with keys:
* - error: Set to 0 if there is no error.
* - request_id: id of the request. You may need it to cancel the request.
* - request_uuid: UUID of the request. You may need it to check the request sate.
* - message: State of the request.
* - mobile_url: An url where you can redirect your user to let them pay. Lydia will
* handle if they already have an account or show the a credit card payment form.
* - order_ref: Only if an order_ref was specified.
*/
$this->url = $result['mobile_url'] ;
return [
'state' => Lydia::WAITING_PAYMENT,
'url' => $this->url,
'request_id' => $result['request_id'],
'request_uuid' => $result['request_uuid'],
] ;
}
/**
* Get the list of required parameters for
* the request.
*
* @return array
*/
protected function shouldHaveParameters() {
return ['recipient', 'amount'] ;
}
/**
* Set the default parameters.
*
* @return void
*/
protected function setDefaultParameters() {
/*
* Vendor token of the business that done the request
* (if it's a request for a business)
*/
$this->setParameter('vendor_token', $this->getLydiaVendorToken()) ;
// recipient type - Allowed values: email, phone
$this->setParameter('type', 'email') ;
/*
* Skip the Lydia confirmation page if browser_success_url is
* also set. This parameter is optional, accepted values are "yes" and "no",
* default is "yes".
*/
$this->setParameter('display_confirmation', 'no') ;
/*
* Defines how the payment should be made: 'lydia' send a payment request to the lydia
* user's phone or send him to a download page if he doesn't have the app. 'cb' redirect
* the user to a classic credit card payment form. 'auto' either 'lydia' or 'cb' depending
* on whether or not a lydia account is associated with this mobile number
*/
$this->setParameter('payment_method', 'cb') ;
// the payer of the payment request by SMS
$this->setParameter('notify', 'no') ;
// send an email to the business owner when the request is accepted by the payer
$this->setParameter('notify_collector', 'no') ;
/*
* duration in seconds after which the payment request becomes invalid. This duration can't
* exceed 7 days (604 800). If the value is up to 604800, the request will automatically
* expire on the 7th day.
*/
$this->setParameter('expire_time', '600') ;
}
/**
* Redirect the client to the Lydia website.
*
* @return mixed
* @throws LydiaException
*/
public function redirect() {
if(is_null($this->url)) {
throw new LydiaException("Impossible to redirect to Lydia's server") ;
}
return $this->lydia()->redirect($this->url) ;
}
/**
* Set the callback when the user is redirected.
*
* @param string $url
*/
public function setFinishCallback(string $url) {
$route = $this->formatCallbackUrl($url) ;
$this->setParameter('confirm_url', $route) ;
$this->setParameter('cancel_url', $route) ;
$this->setParameter('end_mobile_url', $route) ;
$this->setParameter('browser_success_url', $route) ;
$this->setParameter('browser_fail_url', $route) ;
}
/**
* Format the callback URL to be valid
* regarding the Lydia server.
*
* @param string $url
* @return string
*/
private function formatCallbackUrl(string $url) {
return $this->lydia()->formatCallbackUrl($url) ;
}
} | 5c2e971ab0317754a701a9933692bad7123be9c5 | [
"Markdown",
"PHP"
] | 17 | PHP | Pythagus/lydia | 63526b390faa5b1e95b238fe85c30edd84712c10 | eee7f540bac749550a7789148d77ffda191dcd56 | |
refs/heads/master | <repo_name>henrhoi/made-pytorch<file_sep>/made.py
import torch.nn as nn
from linear_masked import LinearMasked
from utils import *
class MADE(nn.Module):
"""
Class implementing MADE for 1 channel image-input based on "MADE: Masked Autoencoder for Distribution Estimation" by <NAME>. al.
"""
def __init__(self, input_dim):
super().__init__()
self.input_dim = input_dim
self.net = nn.Sequential(
LinearMasked(input_dim, input_dim),
nn.ReLU(),
LinearMasked(input_dim, input_dim),
nn.ReLU(),
LinearMasked(input_dim, input_dim)
)
self.apply_masks()
def forward(self, x):
return self.net(x)
def apply_masks(self):
# Set order of masks, i.e. who can make which edges
ordering = np.arange(self.input_dim)
# Create masks
masks = []
masks.append(ordering[:, None] <= ordering[None, :])
masks.append(ordering[:, None] <= ordering[None, :])
masks.append(ordering[:, None] < ordering[None, :])
# Set the masks in all LinearMasked layers
layers = [layer for layer in self.net.modules() if isinstance(layer, LinearMasked)]
for i in range(len(layers)):
layers[i].set_mask(masks[i])
def train_made(train_data, test_data, image_shape, dset_id):
"""
train_data: A (n_train, H, W, 1) uint8 numpy array of binary images with values in {0, 1}
test_data: An (n_test, H, W, 1) uint8 numpy array of binary images with values in {0, 1}
image_shape: (H, W), height and width of the image
dset_id: An identifying number of which dataset is given (1 or 2). Most likely
used to set different hyperparameters for different datasets
Returns
- a (# of training iterations,) numpy array of train_losses evaluated every minibatch
- a (# of epochs + 1,) numpy array of test_losses evaluated once at initialization and after each epoch
- a numpy array of size (100, H, W, 1) of samples with values in {0, 1}
"""
use_cuda = False
device = torch.device('cuda') if use_cuda else None
train_data = torch.from_numpy(
train_data.reshape((train_data.shape[0], train_data.shape[1] * train_data.shape[2]))).float().to(device)
test_data = torch.from_numpy(
test_data.reshape((test_data.shape[0], test_data.shape[1] * test_data.shape[2]))).float().to(device)
def nll_loss(batch, output):
return torch.nn.functional.binary_cross_entropy(torch.sigmoid(output), batch)
H, W = image_shape[0], image_shape[1]
input_dim = H * W
dataset_params = {
'batch_size': 32,
'shuffle': True
}
made = MADE(input_dim).cuda() if use_cuda else MADE(input_dim)
n_epochs = 10
lr = 0.003
train_loader = torch.utils.data.DataLoader(train_data, **dataset_params)
optimizer = torch.optim.Adam(made.parameters(), lr=lr)
init_test_loss = nll_loss(test_data, made(test_data))
train_losses = []
test_losses = [init_test_loss.item()]
for epoch in range(n_epochs):
for batch_x in train_loader:
optimizer.zero_grad()
output = made(batch_x)
loss = nll_loss(batch_x, output)
loss.backward()
optimizer.step()
train_losses.append(loss.item())
test_loss = nll_loss(test_data, made(test_data))
test_losses.append(test_loss.item())
print(f"[{100*(epoch+1)/n_epochs:.2f}%] Epoch {epoch + 1}")
if use_cuda:
torch.cuda.empty_cache()
samples = torch.zeros(size=(100, H * W)).to(device)
made.eval()
with torch.no_grad():
for i in range(H * W):
out = made(samples)
proba = torch.sigmoid(out)
torch.bernoulli(proba[:, i], out=samples[:, i])
return np.array(train_losses), np.array(test_losses), samples.reshape((100, H, W, 1)).detach().cpu().numpy()
def train_and_show_results_shapes_data():
"""
Trains MADE and displays samples and training plot for Shapes dataset
"""
show_results_made(1, 'b', train_made)
def train_and_show_results_mnist_data():
"""
Trains MADE and displays samples and training plot for MNIST dataset
"""
show_results_made(2, 'b', train_made)
<file_sep>/made2d.py
import torch.nn as nn
from linear_masked import LinearMasked
from utils import *
class MADE2D(nn.Module):
"""
Class implementing MADE for 2D input based on "MADE: Masked Autoencoder for Distribution Estimation" by <NAME>. al.
"""
def __init__(self, input_dim, d):
super().__init__()
self.d = d
self.input_dim = input_dim
self.net = nn.Sequential(
LinearMasked(input_dim, input_dim),
nn.ReLU(),
LinearMasked(input_dim, input_dim),
nn.ReLU(),
LinearMasked(input_dim, input_dim)
)
self.apply_masks()
def forward(self, x):
return self.net(x)
def apply_masks(self):
# Set order of masks, i.e. who can make which edges
order_0 = np.concatenate((np.repeat(1, self.d), np.repeat(2, self.d)))
order_1 = np.repeat(1, self.input_dim)
order_2 = np.repeat(1, self.input_dim)
# Create masks
masks = []
masks.append(order_0[:, None] <= order_1[None, :])
masks.append(order_1[:, None] <= order_2[None, :])
masks.append(order_2[:, None] < order_0[None, :])
# Set the masks in all LinearMasked layers
layers = [layer for layer in self.net.modules() if isinstance(layer, LinearMasked)]
for i in range(len(layers)):
layers[i].set_mask(masks[i])
def train_made(train_data, test_data, d, dset_id):
"""
train_data: An (n_train, 2) numpy array of integers in {0, ..., d-1}
test_data: An (n_test, 2) numpy array of integers in {0, .., d-1}
d: The number of possible discrete values for each random variable x1 and x2
dset_id: An identifying number of which dataset is given (1 or 2). Most likely
used to set different hyperparameters for different datasets
Returns
- a (# of training iterations,) numpy array of train_losses evaluated every minibatch
- a (# of epochs + 1,) numpy array of test_losses evaluated once at initialization and after each epoch
- a numpy array of size (d, d) of probabilities (the learned joint distribution)
"""
def make_one_hot(data, num_classes=d):
one_hot = np.zeros((len(data), 2 * d))
one_hot[np.arange(data.shape[0]), data[:, 0]] = 1
one_hot[np.arange(data.shape[0]), d + data[:, 1]] = 1
return torch.from_numpy(one_hot).float()
use_cuda = True
device = torch.device('cuda') if use_cuda else None
train_data = make_one_hot(train_data).to(device)
test_data = make_one_hot(test_data).to(device)
def get_proba(batch, output, d):
output_1 = torch.softmax(output[:, :d], dim=1)
output_2 = torch.softmax(output[:, d:], dim=1)
indices_1 = torch.nonzero(batch[:, :d])
indices_2 = torch.nonzero(batch[:, d:])
return torch.gather(input=output_1, dim=1, index=indices_1[:, [1]]) \
* torch.gather(input=output_2, dim=1, index=indices_2[:, [1]])
def nll_loss(batch, output, d):
proba = get_proba(batch, output, d)
return -torch.mean(torch.log(proba + 1e-8))
made = MADE2D(2 * d, d).cuda() if use_cuda else MADE2D(2 * d, d)
dataset_params = {
'batch_size': 32,
'shuffle': True
}
n_epochs = 50 if dset_id == 2 else 10
lr = 0.0025 if dset_id == 2 else 0.01
train_loader = torch.utils.data.DataLoader(train_data, **dataset_params)
optimizer = torch.optim.Adam(made.parameters(), lr=lr)
init_test_loss = nll_loss(test_data, made(test_data), d)
train_losses = []
test_losses = [init_test_loss.item()]
for epoch in range(n_epochs):
for batch_x in train_loader:
optimizer.zero_grad()
output = made(batch_x)
loss = nll_loss(batch_x, output, d)
loss.backward()
optimizer.step()
train_losses.append(loss.item())
test_loss = nll_loss(torch.tensor(test_data), made(torch.tensor(test_data)), d)
test_losses.append(test_loss.item())
print(f"[{100*(epoch+1)/n_epochs:.2f}%] Epoch {epoch + 1}") if epoch % 2 else None
x_s = make_one_hot(torch.tensor([[i, j] for i in range(d) for j in range(d)])).to(device)
proba = get_proba(x_s, made(x_s), d).reshape(d, d)
return np.array(train_losses), np.array(test_losses), proba.detach().cpu().numpy()
def train_and_show_results_smiley_data():
"""
Trains 2D MADE and displays samples and training plot for smiley distribution
"""
show_results_made(1, 'a', train_made)
def train_and_show_results_geoffrey_hinton_data():
"""
Trains 2D MADE and displays samples and training plot for Geoffrey Hinton distribution
"""
show_results_made(2, 'a', train_made)
<file_sep>/utils.py
import pickle
from os.path import join
from torchvision.utils import make_grid
import matplotlib.pyplot as plt
import numpy as np
# Mixture of logistics
import torch
def mixture_of_logistics_sample_data_1():
count = 1000
rand = np.random.RandomState(0)
samples = 0.4 + 0.1 * rand.randn(count)
data = np.digitize(samples, np.linspace(0.0, 1.0, 20))
split = int(0.8 * len(data))
train_data, test_data = data[:split], data[split:]
return train_data, test_data
def mixture_of_logistics_sample_data_2():
count = 10000
rand = np.random.RandomState(0)
a = 0.3 + 0.1 * rand.randn(count)
b = 0.8 + 0.05 * rand.randn(count)
mask = rand.rand(count) < 0.5
samples = np.clip(a * mask + b * (1 - mask), 0.0, 1.0)
data = np.digitize(samples, np.linspace(0.0, 1.0, 100))
split = int(0.8 * len(data))
train_data, test_data = data[:split], data[split:]
return train_data, test_data
def show_training_plot(train_losses, test_losses, title):
plt.figure()
n_epochs = len(test_losses) - 1
x_train = np.linspace(0, n_epochs, len(train_losses))
x_test = np.arange(n_epochs + 1)
plt.plot(x_train, train_losses, label='train loss')
plt.plot(x_test, test_losses, label='test loss')
plt.legend()
plt.title(title)
plt.xlabel('Epoch')
plt.ylabel('NLL')
plt.show()
def show_distribution_1d(data, distribution, title):
d = len(distribution)
plt.figure()
plt.hist(data, bins=np.arange(d) - 0.5, label='train data', density=True)
x = np.linspace(-0.5, d - 0.5, 1000)
y = distribution.repeat(1000 // d)
plt.plot(x, y, label='learned distribution')
plt.title(title)
plt.xlabel('x')
plt.ylabel('Probability')
plt.legend()
plt.show()
def show_results(dset_type, fn):
if dset_type == 1:
train_data, test_data = mixture_of_logistics_sample_data_1()
d = 20
elif dset_type == 2:
train_data, test_data = mixture_of_logistics_sample_data_2()
d = 100
else:
raise Exception('Invalid dset_type:', dset_type)
train_losses, test_losses, distribution = fn(train_data, test_data, d, dset_type)
assert np.allclose(np.sum(distribution), 1), f'Distribution sums to {np.sum(distribution)} != 1'
print(f'Final Test Loss: {test_losses[-1]:.4f}')
show_training_plot(train_losses, test_losses, f'Dataset {dset_type} Train Plot')
show_distribution_1d(train_data, distribution, f'Dataset {dset_type} Learned Distribution')
# MADE
def load_pickled_data(fname, include_labels=False):
with open(fname, 'rb') as f:
data = pickle.load(f)
train_data, test_data = data['train'], data['test']
if 'mnist.pkl' in fname or 'shapes.pkl' in fname:
# Binarize MNIST and shapes dataset
train_data = (train_data > 127.5).astype('uint8')
test_data = (test_data > 127.5).astype('uint8')
if 'celeb.pkl' in fname:
train_data = train_data[:, :, :, [2, 1, 0]]
test_data = test_data[:, :, :, [2, 1, 0]]
if include_labels:
return train_data, test_data, data['train_labels'], data['test_labels']
return train_data, test_data
# Question 2
def sample_2d_data(image_file, n, d):
from PIL import Image
import itertools
im = Image.open(image_file).resize((d, d)).convert('L')
im = np.array(im).astype('float32')
dist = im / im.sum()
pairs = list(itertools.product(range(d), range(d)))
idxs = np.random.choice(len(pairs), size=n, replace=True, p=dist.reshape(-1))
samples = [pairs[i] for i in idxs]
return dist, np.array(samples)
def show_distribution_2d(true_dist, learned_dist):
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(12, 8))
ax1.imshow(true_dist)
ax1.set_title('True Distribution')
ax1.axis('off')
ax2.imshow(learned_dist)
ax2.set_title('Learned Distribution')
ax2.axis('off')
plt.show()
def show_samples(samples, nrow=10, title='Samples'):
samples = (torch.FloatTensor(samples) / 255).permute(0, 3, 1, 2)
grid_img = make_grid(samples, nrow=nrow)
plt.figure()
plt.title(title)
plt.imshow(grid_img.permute(1, 2, 0))
plt.axis('off')
plt.show()
def show_results_made(dset_type, part, fn):
data_dir = "data"
if part == 'a':
if dset_type == 1:
n, d = 10000, 25
true_dist, data = sample_2d_data(join(data_dir, 'smiley.jpg'), n, d)
elif dset_type == 2:
n, d = 100000, 200
true_dist, data = sample_2d_data(join(data_dir, 'geoffrey-hinton.jpg'), n, d)
else:
raise Exception('Invalid dset_type:', dset_type)
split = int(0.8 * len(data))
train_data, test_data = data[:split], data[split:]
elif part == 'b':
if dset_type == 1:
train_data, test_data = load_pickled_data(join(data_dir, 'shapes.pkl'))
img_shape = (20, 20)
elif dset_type == 2:
train_data, test_data = load_pickled_data(join(data_dir, 'mnist.pkl'))
img_shape = (28, 28)
else:
raise Exception('Invalid dset type:', dset_type)
else:
raise Exception('Invalid part', part)
if part == 'a':
train_losses, test_losses, distribution = fn(train_data, test_data, d, dset_type)
assert np.allclose(np.sum(distribution), 1), f'Distribution sums to {np.sum(distribution)} != 1'
print(f'Final Test Loss: {test_losses[-1]:.4f}')
show_training_plot(train_losses, test_losses, f'Dataset {dset_type} Train Plot)')
show_distribution_2d(true_dist, distribution)
elif part == 'b':
train_losses, test_losses, samples = fn(train_data, test_data, img_shape, dset_type)
samples = samples.astype('float32') * 255
print(f'Final Test Loss: {test_losses[-1]:.4f}')
show_training_plot(train_losses, test_losses, f'Dataset {dset_type} Train Plot')
show_samples(samples)
<file_sep>/mixture_of_logistics.py
from utils import *
def generative_model_histogram(train_data, test_data, d, dset_id):
"""
train_data: An (n_train,) numpy array of integers in {0, ..., d-1}
test_data: An (n_test,) numpy array of integers in {0, .., d-1}
d: The number of possible discrete values for random variable x
dset_id: An identifying number of which dataset is given (1 or 2). Most likely
used to set different hyperparameters for different datasets
Returns
- a (# of training iterations,) numpy array of train_losses evaluated every minibatch
- a (# of epochs + 1,) numpy array of test_losses evaluated once at initialization and after each epoch
- a numpy array of size (d,) of model probabilities
"""
def nll_loss(x, theta):
model = torch.exp(torch.gather(theta, dim=0, index=x)) / torch.exp(theta).sum()
return torch.mean(-torch.log(model))
dataset_params = {
'batch_size': 32,
'shuffle': True
}
theta = torch.zeros(d, dtype=torch.float32, requires_grad=True)
n_epochs = 40
train_loader = torch.utils.data.DataLoader(train_data, **dataset_params)
optimizer = torch.optim.Adam([theta], lr=0.01)
train_losses = []
test_losses = [nll_loss(torch.tensor(test_data), theta).item()]
for epoch in range(n_epochs):
for batch_x in train_loader:
optimizer.zero_grad()
loss = nll_loss(batch_x, theta)
loss.backward()
optimizer.step()
train_losses.append(loss.item())
test_loss = nll_loss(torch.tensor(test_data), theta)
test_losses.append(test_loss.item())
return np.array(train_losses), np.array(test_losses), torch.softmax(theta, dim=0).detach().numpy()
def discretized_mixture_of_logistics(train_data, test_data, d, dset_id):
"""
train_data: An (n_train,) numpy array of integers in {0, ..., d-1}
test_data: An (n_test,) numpy array of integers in {0, .., d-1}
d: The number of possible discrete values for random variable x
dset_id: An identifying number of which dataset is given (1 or 2). Most likely
used to set different hyperparameters for different datasets
Returns
- a (# of training iterations,) numpy array of train_losses evaluated every minibatch
- a (# of epochs + 1,) numpy array of test_losses evaluated once at initialization and after each epoch
- a numpy array of size (d,) of model probabilities
"""
def model(x, pis, means, stds):
x = x.reshape((x.shape[0], 1))
x_plus = x + 0.5
x_minus = x - 0.5
# Handeling edge cases
x_plus[x_plus > d - 1] = torch.tensor(float('inf'), requires_grad=True)
x_minus[x_minus < 0] = torch.tensor(float('-inf'), requires_grad=True)
unweighted_mixtures = torch.sigmoid((x_plus - means) / torch.exp(stds)) - torch.sigmoid(
(x_minus - means) / torch.exp(stds))
model_proba = (unweighted_mixtures * torch.softmax(pis, dim=1)).sum(dim=1, keepdim=False)
return model_proba
def nll_loss(x, pis, means, stds):
model_proba = model(x, pis, means, stds)
return torch.mean(-torch.log(model_proba))
dataset_params = {
'batch_size': 32,
'shuffle': True
}
if dset_id == 2:
pis = torch.tensor([[1., 2., 3., 4.]], requires_grad=True)
means = torch.tensor([[20., 30., 80., 90.]], requires_grad=True)
stds = torch.tensor([[1., 2., 3., 4.]], requires_grad=True)
else:
pis = torch.tensor([[1., 2., 3., 4.]], requires_grad=True)
means = torch.tensor([[1., 2., 3., 4.]], requires_grad=True)
stds = torch.tensor([[1., 2., 3., 4.]], requires_grad=True)
n_epochs = 20
train_loader = torch.utils.data.DataLoader(train_data, **dataset_params)
optimizer = torch.optim.Adam([pis, means, stds], lr=0.01)
train_losses = []
test_losses = [nll_loss(torch.tensor(test_data), pis, means, stds).item()]
for epoch in range(n_epochs):
for batch_x in train_loader:
optimizer.zero_grad()
loss = nll_loss(batch_x, pis, means, stds)
loss.backward()
optimizer.step()
train_losses.append(loss.item())
test_loss = nll_loss(torch.tensor(test_data), pis, means, stds)
test_losses.append(test_loss.item())
x_s = torch.tensor([i for i in range(d)])
model_probabilities = model(x_s, pis, means, stds)
return np.array(train_losses), np.array(test_losses), model_probabilities.detach().numpy()
if __name__ == '__main__':
show_results(dset_type=1, fn=generative_model_histogram)
show_results(dset_type=2, fn=discretized_mixture_of_logistics)
<file_sep>/linear_masked.py
import torch
import torch.nn as nn
class LinearMasked(nn.Linear):
"""
Class implementing nn.Linear with mask
"""
def __init__(self, in_features, out_features, bias=True):
super().__init__(in_features, out_features, bias)
self.register_buffer('mask', torch.ones(out_features, in_features))
def set_mask(self, mask):
self.mask.data.copy_(torch.from_numpy(mask.astype(np.uint8).T))
def forward(self, x):
return torch.nn.functional.linear(x, self.mask * self.weight, self.bias)
<file_sep>/README.md
# MADE (Masked Autoencoder Distribution Estimation) in PyTorch
PyTorch implementations of MADE (Masked Autoencoder Distribution Estimation) based on [MADE: Masked Autoencoder for Distribution Estimation](https://arxiv.org/abs/1502.03509) by Germain et. al. and autoregressive models.
## Models
**MADE - Fitting 2D Data:**
Implemented a [MADE](https://arxiv.org/abs/1502.03509) model through maximum likelihood to represent <img src="https://render.githubusercontent.com/render/math?math=p(x_0,x_1)"> on the given datasets, with any autoregressive ordering.
**MADE:**
Implemented a MADE model on the binary image datasets Shape and MNIST. Given some binary image of height <img src="https://render.githubusercontent.com/render/math?math=H"> and width <img src="https://render.githubusercontent.com/render/math?math=W">, we can represent image <img src="https://render.githubusercontent.com/render/math?math=x\in \{0, 1\}^{H\times W}"> as a flattened binary vector <img src="https://render.githubusercontent.com/render/math?math=x\in \{0, 1\}^{HW}"> to input into MADE to model <img src="https://render.githubusercontent.com/render/math?math=p_\theta(x) = \prod_{i=1}^{HW} p_\theta(x_i|x_{ < i})">.
Illustration of MADE-model:

**Fitting a histogram:**
Let <img src="https://render.githubusercontent.com/render/math?math=\theta = (\theta_0, \dots, \theta_{d-1}) \in \mathbb{R}^d"> and define the model <img src="https://render.githubusercontent.com/render/math?math=p_\theta(x) = \dfrac{e^{\theta_x}}{\sum_{x'}e^{\theta_{x'}}}">
Fit <img src="https://render.githubusercontent.com/render/math?math=p_\theta"> with maximum likelihood via stochastic gradient descent on the training set, using <img src="https://render.githubusercontent.com/render/math?math=\theta"> initialized to zero.
**Discretized Mixture of Logistics:**
Let us model <img src="https://render.githubusercontent.com/render/math?math=p_\theta(x)"> as a **discretized** mixture of 4 logistics such that <img src="https://render.githubusercontent.com/render/math?math=p_\theta(x) = \sum_{i=1}^4 \pi_i[\sigma((x+0.5 - \mu_i)/s_i) - \sigma((x-0.5-\mu_i)/s_i)]">
For the edge case of when <img src="https://render.githubusercontent.com/render/math?math=x = 0">, we replace <img src="https://render.githubusercontent.com/render/math?math=x-0.5"> by <img src="https://render.githubusercontent.com/render/math?math=-\infty">, and for <img src="https://render.githubusercontent.com/render/math?math=x = d-1">, we replace <img src="https://render.githubusercontent.com/render/math?math=x+0.5"> by <img src="https://render.githubusercontent.com/render/math?math=\infty">.
One may find the [PixelCNN++](https://arxiv.org/abs/1701.05517) helpful for more information on discretized mixture of logistics.
## Results and samples
| Model | Dataset | Result/Samples |
|:---:|:---:|:---:|
| MADE 2D (Smiley) |  |  |
| MADE 2D (<NAME>) |  |  |
| MADE (Shapes) |  |  |
| MADE (MNIST) |  |  |
| Simple histogram |  |  |
| Discretized Mixture of Logistics|  |  |
| 2e0e504d9364c93952465de806018d5659cd67e4 | [
"Markdown",
"Python"
] | 6 | Python | henrhoi/made-pytorch | edcaf6fe0f84c1a5d5a815e07bb6fec0148bb80a | 678d1a466f0a6ed5e7a667dcf6c56b15f257f2a0 | |
refs/heads/main | <repo_name>SunHailang/LeranOpenGL<file_sep>/LeranOpenGL/OpenGLAdvanced/src/Mesh.cpp
#include "Mesh.h"
#include <iostream>
#include "GL/glew.h"
#include "GLFW/glfw3.h"
#include "ShaderProgram.h"
#include "GLDebug.h"
Mesh::Mesh(std::vector<Vertex> _vertices, std::vector<unsigned int> _indices, std::vector<Texture> _textures)
:vertices(_vertices), indices(_indices), textures(_textures)
{
SetupMesh();
}
Mesh::~Mesh()
{
//GLCall(glDeleteVertexArrays(1, &VAO));
//GLCall(glDeleteBuffers(1, &VBO));
//GLCall(glDeleteBuffers(1, &EBO));
}
void Mesh::Draw(ShaderProgram *shaderProgram) const
{
unsigned int textureIndex = 0;
for (unsigned int i = 0; i < textures.size(); i++)
{
glActiveTexture(GL_TEXTURE0 + textureIndex); // 在绑定之前激活相应的纹理单元
std::string name = textures.at(i).type.c_str();
unsigned int id = textures.at(i).id;
shaderProgram->SetUniform1i(name, textureIndex);
textureIndex++;
glBindTexture(GL_TEXTURE_2D, textures[i].id);
}
// 绘制网格
glBindVertexArray(VAO);
glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_INT, 0);
glBindVertexArray(0);
}
void Mesh::SetupMesh()
{
GLCall(glGenVertexArrays(1, &VAO));
GLCall(glGenBuffers(1, &VBO));
GLCall(glGenBuffers(1, &EBO));
GLCall(glBindVertexArray(VAO));
GLCall(glBindBuffer(GL_ARRAY_BUFFER, VBO));
GLCall(glBufferData(GL_ARRAY_BUFFER, vertices.size() * sizeof(Vertex), &vertices[0], GL_STATIC_DRAW));
GLCall(glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, EBO));
GLCall(glBufferData(GL_ELEMENT_ARRAY_BUFFER, indices.size() * sizeof(unsigned int), &indices[0], GL_STATIC_DRAW));
// 顶点位置
GLCall(glEnableVertexAttribArray(0));
GLCall(glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, sizeof(Vertex), (void*)0));
// 顶点法线
GLCall(glEnableVertexAttribArray(1));
GLCall(glVertexAttribPointer(1, 3, GL_FLOAT, GL_FALSE, sizeof(Vertex), (void*)offsetof(Vertex, Normal)));
// 顶点纹理坐标
GLCall(glEnableVertexAttribArray(2));
GLCall(glVertexAttribPointer(2, 2, GL_FLOAT, GL_FALSE, sizeof(Vertex), (void*)offsetof(Vertex, TexCoords)));
GLCall(glBindVertexArray(0));
}
<file_sep>/LeranOpenGL/OpenGL/src/Application.cpp
#include <GL\glew.h>
#include <GLFW\glfw3.h>
#include <iostream>
#include "Renderer.h"
#include "VertexBuffer.h"
#include "IndexBuffer.h"
#include "VertexArray.h"
#include "VertexBufferLayout.h"
#include "ShaderProgram.h"
#include "Texture.h"
#include "glm/glm.hpp"
#include <glm/gtc/matrix_transform.hpp>
#include <glm/gtc/type_ptr.hpp>
#include "imgui/imgui.h"
#include "imgui/imgui_impl_glfw.h"
#include "imgui/imgui_impl_opengl3.h"
#include "Camera.h"
#include "Time.h"
#define SCREEN_WIDTH 960
#define SCREEN_HEIGHT 540
void framebuffer_size_callback(GLFWwindow* window, int width, int height);
void mouse_callback(GLFWwindow* window, double xpos, double ypos);
void scroll_callback(GLFWwindow* window, double xoffset, double yoffset);
void processInput(GLFWwindow *window);
// camera
Camera camera(glm::vec3(0.0f, 0.0f, 3.0f));
float lastX = SCREEN_WIDTH / 2.0f;
float lastY = SCREEN_HEIGHT / 2.0f;
bool firstMouse = true;
// timing
float deltaTime = 0.0f; // time between current frame and last frame
float lastFrame = 0.0f;
int main(void)
{
GLFWwindow* window;
if (!glfwInit())
return -1;
// GL 3.0 + GLSL 130
const char* glsl_version = "#version 130";
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
window = glfwCreateWindow(SCREEN_WIDTH, SCREEN_HEIGHT, "Hello World", NULL, NULL);
if (!window)
{
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
// Enable vsync
glfwSwapInterval(1);
glfwSetFramebufferSizeCallback(window, framebuffer_size_callback);
glfwSetCursorPosCallback(window, mouse_callback);
glfwSetScrollCallback(window, scroll_callback);
// tell GLFW to capture our mouse
//glfwSetInputMode(window, GLFW_CURSOR, GLFW_CURSOR_DISABLED);
if (glewInit() != GLEW_OK)
{
std::cout << "glew init failed." << std::endl;
glfwTerminate();
return -1;
}
std::cout << "[OpenGL Version]: " << glfwGetVersionString() << std::endl;
{
float positions[] = {
// positions // normals // texture coords
-0.5f, -0.5f, -0.5f, 0.0f, 0.0f, -1.0f, 0.0f, 0.0f,
0.5f, -0.5f, -0.5f, 0.0f, 0.0f, -1.0f, 1.0f, 0.0f,
0.5f, 0.5f, -0.5f, 0.0f, 0.0f, -1.0f, 1.0f, 1.0f,
0.5f, 0.5f, -0.5f, 0.0f, 0.0f, -1.0f, 1.0f, 1.0f,
-0.5f, 0.5f, -0.5f, 0.0f, 0.0f, -1.0f, 0.0f, 1.0f,
-0.5f, -0.5f, -0.5f, 0.0f, 0.0f, -1.0f, 0.0f, 0.0f,
-0.5f, -0.5f, 0.5f, 0.0f, 0.0f, 1.0f, 0.0f, 0.0f,
0.5f, -0.5f, 0.5f, 0.0f, 0.0f, 1.0f, 1.0f, 0.0f,
0.5f, 0.5f, 0.5f, 0.0f, 0.0f, 1.0f, 1.0f, 1.0f,
0.5f, 0.5f, 0.5f, 0.0f, 0.0f, 1.0f, 1.0f, 1.0f,
-0.5f, 0.5f, 0.5f, 0.0f, 0.0f, 1.0f, 0.0f, 1.0f,
-0.5f, -0.5f, 0.5f, 0.0f, 0.0f, 1.0f, 0.0f, 0.0f,
-0.5f, 0.5f, 0.5f, -1.0f, 0.0f, 0.0f, 1.0f, 0.0f,
-0.5f, 0.5f, -0.5f, -1.0f, 0.0f, 0.0f, 1.0f, 1.0f,
-0.5f, -0.5f, -0.5f, -1.0f, 0.0f, 0.0f, 0.0f, 1.0f,
-0.5f, -0.5f, -0.5f, -1.0f, 0.0f, 0.0f, 0.0f, 1.0f,
-0.5f, -0.5f, 0.5f, -1.0f, 0.0f, 0.0f, 0.0f, 0.0f,
-0.5f, 0.5f, 0.5f, -1.0f, 0.0f, 0.0f, 1.0f, 0.0f,
0.5f, 0.5f, 0.5f, 1.0f, 0.0f, 0.0f, 1.0f, 0.0f,
0.5f, 0.5f, -0.5f, 1.0f, 0.0f, 0.0f, 1.0f, 1.0f,
0.5f, -0.5f, -0.5f, 1.0f, 0.0f, 0.0f, 0.0f, 1.0f,
0.5f, -0.5f, -0.5f, 1.0f, 0.0f, 0.0f, 0.0f, 1.0f,
0.5f, -0.5f, 0.5f, 1.0f, 0.0f, 0.0f, 0.0f, 0.0f,
0.5f, 0.5f, 0.5f, 1.0f, 0.0f, 0.0f, 1.0f, 0.0f,
-0.5f, -0.5f, -0.5f, 0.0f, -1.0f, 0.0f, 0.0f, 1.0f,
0.5f, -0.5f, -0.5f, 0.0f, -1.0f, 0.0f, 1.0f, 1.0f,
0.5f, -0.5f, 0.5f, 0.0f, -1.0f, 0.0f, 1.0f, 0.0f,
0.5f, -0.5f, 0.5f, 0.0f, -1.0f, 0.0f, 1.0f, 0.0f,
-0.5f, -0.5f, 0.5f, 0.0f, -1.0f, 0.0f, 0.0f, 0.0f,
-0.5f, -0.5f, -0.5f, 0.0f, -1.0f, 0.0f, 0.0f, 1.0f,
-0.5f, 0.5f, -0.5f, 0.0f, 1.0f, 0.0f, 0.0f, 1.0f,
0.5f, 0.5f, -0.5f, 0.0f, 1.0f, 0.0f, 1.0f, 1.0f,
0.5f, 0.5f, 0.5f, 0.0f, 1.0f, 0.0f, 1.0f, 0.0f,
0.5f, 0.5f, 0.5f, 0.0f, 1.0f, 0.0f, 1.0f, 0.0f,
-0.5f, 0.5f, 0.5f, 0.0f, 1.0f, 0.0f, 0.0f, 0.0f,
-0.5f, 0.5f, -0.5f, 0.0f, 1.0f, 0.0f, 0.0f, 1.0f
};
// world space positions of our cubes
glm::vec3 cubePositions[] = {
glm::vec3(0.0f, 0.0f, 0.0f),
glm::vec3(2.0f, 5.0f, -15.0f),
glm::vec3(-1.5f, -2.2f, -2.5f),
glm::vec3(-3.8f, -2.0f, -12.3f),
glm::vec3(2.4f, -0.4f, -3.5f),
glm::vec3(-1.7f, 3.0f, -7.5f),
glm::vec3(1.3f, -2.0f, -2.5f),
glm::vec3(1.5f, 2.0f, -2.5f),
glm::vec3(1.5f, 0.2f, -1.5f),
glm::vec3(-1.3f, 1.0f, -1.5f)
};
GLCall(glEnable(GL_BLEND));
GLCall(glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA));
GLCall(glEnable(GL_DEPTH_TEST));
VertexArray va;
VertexBuffer vb(positions, sizeof(positions));
VertexBufferLayout layout;
layout.Push<float>(3);
layout.Push<float>(3);
layout.Push<float>(2);
va.AddBuffer(vb, layout);
//IndexBuffer ib(indices, 0);
VertexArray lightVAO;
VertexBufferLayout lightLayout;
lightLayout.Push<float>(3);
lightLayout.Push<float>(3);
lightLayout.Push<float>(2);
lightVAO.AddBuffer(vb, lightLayout);
lightVAO.Unbind();
ShaderProgram program("res/shaders/Basic.shader");
program.Bind();
program.SetUniform4f("u_Color", 0.2f, 0.3f, 0.3f, 1.0f);
//Texture texture("res/textures/log.png");
//texture.Bind();
program.SetUniform1i("u_LightTexture", 0);
//ShaderProgram lithtProgram("res/shaders/Light.shader");
ShaderProgram lithtProgram("res/shaders/Material.shader");
Texture tex("res/textures/container2.png");
tex.Bind();
lithtProgram.Bind();
Texture tex1("res/textures/container2_specular.png");
tex1.Bind();
//lithtProgram.SetUniform1i("material.diffuse", 0);
//lithtProgram.SetUniform1i("material.specular", 1);
va.Unbind();
vb.Unbind();
//ib.Unbind();
program.Unbind();
lithtProgram.Unbind();
Renderer renderer;
ImGui::CreateContext();
ImGuiIO& io = ImGui::GetIO(); (void)io;
//io.ConfigFlags |= ImGuiConfigFlags_NavEnableKeyboard; // Enable Keyboard Controls
//io.ConfigFlags |= ImGuiConfigFlags_NavEnableGamepad; // Enable Gamepad Controls
// Setup Dear ImGui style
//ImGui::StyleColorsDark();
ImGui::StyleColorsClassic();
// Setup Platform/Renderer bindings
ImGui_ImplGlfw_InitForOpenGL(window, true);
ImGui_ImplOpenGL3_Init(glsl_version);
float specularStr = 32.0f;
glm::vec3 translation(0.0f, -0.5f, -4.0f);
while (!glfwWindowShouldClose(window))
{
/* renderer here */
renderer.Clear();
float currentFrame = (float)glfwGetTime();
deltaTime = currentFrame - lastFrame;
lastFrame = currentFrame;
processInput(window);
// Start the Dear ImGui frame
ImGui_ImplOpenGL3_NewFrame();
ImGui_ImplGlfw_NewFrame();
ImGui::NewFrame();
program.Bind();
//renderer.Draw(va, ib, program);
// create transformations
glm::mat4 model = glm::mat4(1.0f); // make sure to initialize matrix to identity matrix first
glm::mat4 view = glm::mat4(1.0f);
glm::mat4 projection = glm::mat4(1.0f);
glm::vec3 lightPos(translation.x, translation.y, translation.z);
model = glm::translate(model, lightPos);
model = glm::scale(model, glm::vec3(0.3f));
model = glm::rotate(model, glm::radians(-55.0f), glm::vec3(0.0f, 1.0f, 0.0f));
//view = glm::translate(view, glm::vec3(0.0f, 0.0f, -3.0f));
//view = glm::translate(view, translation);
projection = glm::perspective(glm::radians(camera.Zoom), (float)SCREEN_WIDTH / (float)SCREEN_HEIGHT, 0.1f, 100.0f);
//projection = glm::ortho(-1.0f, 1.0f, -1.0f, 1.0f, -1.0f, 1.0f);
float radius = 20.0f;
float camX = sin((float)glfwGetTime()) * radius;
float camZ = cos((float)glfwGetTime()) * radius;
view = camera.GetViewMatrix();
//view = glm::lookAt(glm::vec3(camX, 0.0, camZ), glm::vec3(0.0, 0.0, 0.0), glm::vec3(0.0, 1.0, 0.0));
//view = glm::translate(glm::mat4(1.0f), translation);
glm::mat4 mvp = projection * view * model;
program.SetUniformMat4f("u_MVP", mvp);
va.Bind();
glDrawArrays(GL_TRIANGLES, 0, 36);
lithtProgram.Bind();
lithtProgram.SetUniform3f("objectColor", 1.0f, 0.5f, 0.31f);
lithtProgram.SetUniform3f("lightColor", 1.0f, 1.0f, 1.0f);
glm::vec3 cubePos(0.2f, -2.5f, -3.0f);
glm::mat4 lightmodel = glm::mat4(1.0f);
//glm::mat4 lightprojection = glm::perspective(glm::radians(camera.Zoom), (float)SCREEN_WIDTH / (float)SCREEN_HEIGHT, 0.1f, 100.0f);
lightmodel = glm::translate(lightmodel, cubePos);
lightmodel = glm::scale(lightmodel, glm::vec3(3.0f));
//lightmodel = glm::rotate(lightmodel, glm::radians(-30.0f), glm::vec3(0.0f, 1.0f, 0.0f));
//glm::mat4 lightMVP = projection * view * lightmodel;
lithtProgram.SetUniformMat4f("projection", projection);
lithtProgram.SetUniformMat4f("view", view);
lithtProgram.SetUniformMat4f("model", lightmodel);
lithtProgram.SetUniform3f("lightPos", lightPos);
lithtProgram.SetUniform3f("viewPos", camera.Position);
glm::vec3 lightColor(1.0f);
//lightColor.x = sin(glfwGetTime() * 2.0f);
//lightColor.y = sin(glfwGetTime() * 0.7f);
//lightColor.z = sin(glfwGetTime() * 1.3f);
glm::vec3 diffuseColor = lightColor * glm::vec3(0.5f); // 降低影响
glm::vec3 ambientColor = diffuseColor * glm::vec3(0.2f); // 很低的影响
lithtProgram.SetUniform3f("material.ambient", 1.0f, 0.5f, 0.31f);
lithtProgram.SetUniform3f("material.diffuse", 1.0f, 0.5f, 0.31f); // 将光照调暗了一些以搭配场景
lithtProgram.SetUniform3f("material.specular", 0.5f, 0.5f, 0.5f);
//lithtProgram.SetUniform3f("light.ambient", ambientColor);
//lithtProgram.SetUniform3f("light.diffuse", diffuseColor); // 将光照调暗了一些以搭配场景
//lithtProgram.SetUniform3f("light.specular", 1.0f, 1.0f, 1.0f);
lithtProgram.SetUniform1f("material.shininess", specularStr);
tex.Bind();
tex1.Bind();
lightVAO.Bind();
glDrawArrays(GL_TRIANGLES, 0, 36);
// 2. Show a simple window that we create ourselves.
// We use a Begin/End pair to created a named window.
{
ImGui::Begin("Application");
ImGui::SliderFloat3("TranslationX", &translation.x, -10.0f, 10.0f);
ImGui::SliderFloat("specular", &specularStr, 8.0f, 256.0f);
ImGui::Text("Application average %.3f ms/frame (%.1f FPS)", 1000.0f / ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
ImGui::End();
}
// Rendering
ImGui::Render();
ImGui_ImplOpenGL3_RenderDrawData(ImGui::GetDrawData());
glfwSwapBuffers(window);
glfwPollEvents();
}
}
// Cleanup
ImGui_ImplOpenGL3_Shutdown();
ImGui_ImplGlfw_Shutdown();
ImGui::DestroyContext();
glfwDestroyWindow(window);
glfwTerminate();
return 0;
}
// process all input: query GLFW whether relevant keys are pressed/released this frame and react accordingly
// ---------------------------------------------------------------------------------------------------------
void processInput(GLFWwindow *window)
{
if (glfwGetKey(window, GLFW_KEY_ESCAPE) == GLFW_PRESS)
glfwSetWindowShouldClose(window, true);
if (glfwGetKey(window, GLFW_KEY_W) == GLFW_PRESS)
camera.ProcessKeyboard(FORWARD, deltaTime);
if (glfwGetKey(window, GLFW_KEY_S) == GLFW_PRESS)
camera.ProcessKeyboard(BACKWARD, deltaTime);
if (glfwGetKey(window, GLFW_KEY_A) == GLFW_PRESS)
camera.ProcessKeyboard(LEFT, deltaTime);
if (glfwGetKey(window, GLFW_KEY_D) == GLFW_PRESS)
camera.ProcessKeyboard(RIGHT, deltaTime);
}
// glfw: whenever the window size changed (by OS or user resize) this callback function executes
// ---------------------------------------------------------------------------------------------
void framebuffer_size_callback(GLFWwindow* window, int width, int height)
{
// make sure the viewport matches the new window dimensions; note that width and
// height will be significantly larger than specified on retina displays.
glViewport(0, 0, width, height);
}
// glfw: whenever the mouse moves, this callback is called
// -------------------------------------------------------
void mouse_callback(GLFWwindow* window, double xpos, double ypos)
{
if (firstMouse)
{
lastX = (float)xpos;
lastY = (float)ypos;
firstMouse = false;
}
float xoffset = (float)xpos - lastX;
float yoffset = lastY - (float)ypos; // reversed since y-coordinates go from bottom to top
lastX = (float)xpos;
lastY = (float)ypos;
//camera.ProcessMouseMovement(xoffset, yoffset);
}
// glfw: whenever the mouse scroll wheel scrolls, this callback is called
// ----------------------------------------------------------------------
void scroll_callback(GLFWwindow* window, double xoffset, double yoffset)
{
camera.ProcessMouseScroll((float)yoffset);
}<file_sep>/LeranOpenGL/OpenGLAdvanced/src/Application.cpp
#include <iostream>
#include <vector>
#include "GL/glew.h"
#include "GLFW/glfw3.h"
#include <glm/glm.hpp>
#include <glm/gtc/matrix_transform.hpp>
#include <glm/gtc/type_ptr.hpp>
#include "imgui/imgui.h"
#include "imgui/imgui_impl_glfw.h"
#include "imgui/imgui_impl_opengl3.h"
#include "GLDebug.h"
#include "Mesh.h"
#include "ShaderProgram.h"
#include "Texture2D.h"
#include "Model.h"
#include "Camera.h"
#include "TextureCube.h"
void glfwFramebufferSizeCallback(GLFWwindow *window, int width, int height);
void mouse_callback(GLFWwindow* window, double xpos, double ypos);
void scroll_callback(GLFWwindow* window, double xoffset, double yoffset);
void processInput(GLFWwindow *window);
const int SCR_WIDTH = 800;
const int SCR_HEIGHT = 600;
// camera
Camera camera(glm::vec3(0.0f, 0.0f, 3.0f));
float lastX = SCR_WIDTH / 2.0f;
float lastY = SCR_HEIGHT / 2.0f;
bool firstMouse = true;
// timing
float deltaTime = 0.0f;
float lastFrame = 0.0f;
int main()
{
GLFWwindow *window;
if (glfwInit() != GL_TRUE)
{
std::cout << "Failed to glfwInit." << std::endl;
return -1;
}
const char* glsl_version = "#version 130";
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
window = glfwCreateWindow(SCR_WIDTH, SCR_HEIGHT, "LeranOpenGL", nullptr, nullptr);
if (window == nullptr)
{
std::cout << "Failed to Create Window." << std::endl;
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
glfwSwapInterval(1);
if (glewInit() != GLEW_OK)
{
std::cout << "Failed to glew Init." << std::endl;
glfwTerminate();
return -1;
}
std::cout << "OpenGL Version: " << glfwGetVersionString() << std::endl;
glViewport(0, 0, SCR_WIDTH, SCR_HEIGHT);
glfwSetFramebufferSizeCallback(window, glfwFramebufferSizeCallback);
glfwSetCursorPosCallback(window, mouse_callback);
glfwSetScrollCallback(window, scroll_callback);
// tell GLFW to capture our mouse
//glfwSetInputMode(window, GLFW_CURSOR, GLFW_CURSOR_DISABLED);
{
GLCall(glEnable(GL_BLEND));
GLCall(glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA));
GLCall(glEnable(GL_DEPTH_TEST));
glEnable(GL_CULL_FACE);
glCullFace(GL_BACK);
glFrontFace(GL_CCW);
// 加载 shader
ShaderProgram lightShaderProgram("res/shaders/Light.shader");
ShaderProgram shaderProgram("res/shaders/Basic.shader");
shaderProgram.Bind();
//shaderProgram.SetUniform1i("ourTexture1", 0);
//shaderProgram.SetUniform1i("ourTexture2", 1);
Model ourModel("res/models/nanosuit/nanosuit.obj");
Model lightCube("res/models/cube/cube.obj");
float transparentVertices[] = {
// positions // texture Coords (swapped y coordinates because texture is flipped upside down)
0.0f, 0.5f, 0.0f, 0.0f, 0.0f,
0.0f, -0.5f, 0.0f, 0.0f, 1.0f,
1.0f, -0.5f, 0.0f, 1.0f, 1.0f,
0.0f, 0.5f, 0.0f, 0.0f, 0.0f,
1.0f, -0.5f, 0.0f, 1.0f, 1.0f,
1.0f, 0.5f, 0.0f, 1.0f, 0.0f
};
// transparent VAO
unsigned int transparentVAO, transparentVBO;
glGenVertexArrays(1, &transparentVAO);
glGenBuffers(1, &transparentVBO);
glBindVertexArray(transparentVAO);
glBindBuffer(GL_ARRAY_BUFFER, transparentVBO);
glBufferData(GL_ARRAY_BUFFER, sizeof(transparentVertices), transparentVertices, GL_STATIC_DRAW);
glEnableVertexAttribArray(0);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 5 * sizeof(float), (void*)0);
glEnableVertexAttribArray(1);
glVertexAttribPointer(1, 2, GL_FLOAT, GL_FALSE, 5 * sizeof(float), (void*)(3 * sizeof(float)));
glBindVertexArray(0);
std::vector<glm::vec3> vegetation
{
glm::vec3(-1.5f, 0.0f, -0.48f),
glm::vec3(1.5f, 0.0f, 0.51f),
glm::vec3(0.0f, 0.0f, 0.7f),
glm::vec3(-0.3f, 0.0f, -2.3f),
glm::vec3(0.5f, 0.0f, -0.6f)
};
ShaderProgram grassShaderProgram("res/shaders/Grass.shader");
grassShaderProgram.Bind();
Texture2D grass("res/textures/grass.png");
grassShaderProgram.SetUniform1i("ourTexture", 0);
#pragma region SkyBox 加载
ShaderProgram skyboxShaderProgram("res/shaders/SkyBox.shader");
float skyboxVertices[] = {
// positions
-70.0f, 70.0f, -70.0f,
-70.0f, -70.0f, -70.0f,
70.0f, -70.0f, -70.0f,
70.0f, -70.0f, -70.0f,
70.0f, 70.0f, -70.0f,
-70.0f, 70.0f, -70.0f, //
-70.0f, -70.0f, 7.00f,
-70.0f, -70.0f, -70.0f,
-70.0f, 70.0f, -70.0f,
-70.0f, 70.0f, -70.0f,
-70.0f, 70.0f, 70.0f,
-70.0f, -70.0f, 70.0f,
70.0f, -70.0f, -70.0f,
70.0f, -70.0f, 70.0f,
70.0f, 70.0f, 70.0f,
70.0f, 70.0f, 70.0f,
70.0f, 70.0f, -70.0f,
70.0f, -70.0f, -70.0f,
-70.0f, -70.0f, 70.0f,
-70.0f, 70.0f, 70.0f,
70.0f, 70.0f, 70.0f,
70.0f, 70.0f, 70.0f,
70.0f, -70.0f, 70.0f,
-70.0f, -70.0f, 70.0f,
-70.0f, 70.0f, -70.0f,
70.0f, 70.0f, -70.0f,
70.0f, 70.0f, 70.0f,
70.0f, 70.0f, 70.0f,
-70.0f, 70.0f, 70.0f,
-70.0f, 70.0f, -70.0f,
-70.0f, -70.0f, -70.0f,
-70.0f, -70.0f, 70.0f,
70.0f, -70.0f, -70.0f,
70.0f, -70.0f, -70.0f,
-70.0f, -70.0f, 70.0f,
70.0f, -70.0f, 70.0f
};
// skybox VAO
unsigned int skyboxVAO, skyboxVBO;
glGenVertexArrays(1, &skyboxVAO);
glGenBuffers(1, &skyboxVBO);
glBindVertexArray(skyboxVAO);
glBindBuffer(GL_ARRAY_BUFFER, skyboxVBO);
glBufferData(GL_ARRAY_BUFFER, sizeof(skyboxVertices), &skyboxVertices, GL_STATIC_DRAW);
glEnableVertexAttribArray(0);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 3 * sizeof(float), (void*)0);
glBindVertexArray(0);
std::vector<std::string> faces
{
"res/textures/skybox/skybox/right.jpg",
"res/textures/skybox/skybox/left.jpg",
"res/textures/skybox/skybox/top.jpg",
"res/textures/skybox/skybox/bottom.jpg",
"res/textures/skybox/skybox/front.jpg",
"res/textures/skybox/skybox/back.jpg"
};
TextureCube textureCube(faces);
skyboxShaderProgram.Bind();
skyboxShaderProgram.SetUniform1i("skybox", 0);
#pragma endregion
//tex2D.Unbind();
shaderProgram.Unbind();
ImGui::CreateContext();
ImGuiIO& io = ImGui::GetIO(); (void)io;
//io.ConfigFlags |= ImGuiConfigFlags_NavEnableKeyboard; // Enable Keyboard Controls
//io.ConfigFlags |= ImGuiConfigFlags_NavEnableGamepad; // Enable Gamepad Controls
// Setup Dear ImGui style
//ImGui::StyleColorsDark();
ImGui::StyleColorsClassic();
// Setup Platform/Renderer bindings
ImGui_ImplGlfw_InitForOpenGL(window, true);
ImGui_ImplOpenGL3_Init(glsl_version);
float rotation = 0;
glm::vec3 lightPos = glm::vec3(-0.5f, 1.0f, 0.0f);
while (!glfwWindowShouldClose(window))
{
// render
// ------
glClearColor(0.1f, 0.1f, 0.1f, 1.0f);
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
// Start the Dear ImGui frame
ImGui_ImplOpenGL3_NewFrame();
ImGui_ImplGlfw_NewFrame();
ImGui::NewFrame();
// per-frame time logic
// --------------------
float currentFrame = (float)glfwGetTime();
deltaTime = currentFrame - lastFrame;
lastFrame = currentFrame;
// input
// -----
processInput(window);
glm::mat4 projection = glm::perspective(glm::radians(camera.Zoom), (float)SCR_WIDTH / (float)SCR_HEIGHT, 0.1f, 100.0f);
glm::mat4 view = camera.GetViewMatrix();
// 绘制天空盒
glDepthFunc(GL_LEQUAL); // change depth function so depth test passes when values are equal to depth buffer's content
skyboxShaderProgram.Bind();
//view = glm::mat4(glm::mat3(camera.GetViewMatrix())); // remove translation from the view matrix
skyboxShaderProgram.SetUniformMat4f("view", view);
skyboxShaderProgram.SetUniformMat4f("projection", projection);
// skybox cube
glBindVertexArray(skyboxVAO);
textureCube.Bind();
glDrawArrays(GL_TRIANGLES, 0, 36);
glBindVertexArray(0);
glDepthFunc(GL_LESS); // set depth function back to default
// 绘制一个光源
lightShaderProgram.Bind();
lightShaderProgram.SetUniformMat4f("projection", projection);
lightShaderProgram.SetUniformMat4f("view", view);
glm::mat4 lightModel = glm::mat4(1.0f);
lightModel = glm::translate(lightModel, lightPos); // translate it down so it's at the center of the scene
lightModel = glm::scale(lightModel, glm::vec3(0.1f)); // it's a bit too big for our scene, so scale it down
lightModel = glm::rotate(lightModel, glm::radians(rotation), glm::vec3(0.0f, 1.0f, 0.0f));
lightShaderProgram.SetUniformMat4f("model", lightModel);
lightCube.Draw(&shaderProgram);
// 绘制3D模型
shaderProgram.Bind();
// view/projection transformations
shaderProgram.SetUniformMat4f("projection", projection);
shaderProgram.SetUniformMat4f("view", view);
// render the loaded model
glm::mat4 model = glm::mat4(1.0f);
model = glm::translate(model, glm::vec3(0.0f, -3.0f, -8.0f)); // translate it down so it's at the center of the scene
model = glm::scale(model, glm::vec3(0.3f)); // it's a bit too big for our scene, so scale it down
model = glm::rotate(model, glm::radians(rotation), glm::vec3(0.0f, 1.0f, 0.0f));
shaderProgram.SetUniformMat4f("model", model);
float x = sin(currentFrame);
shaderProgram.SetUniform3f("lightColor", glm::vec3(1.0f));
shaderProgram.SetUniform3f("lightPos", lightPos);
shaderProgram.SetUniform3f("lightAmbient", glm::vec3(0.05f, 0.05f, 0.05f));
shaderProgram.SetUniform3f("lightDiffuse", glm::vec3(1.0f, 0.5f, 0.31f));
shaderProgram.SetUniform3f("lightSpecular", glm::vec3(0.5f, 0.5f, 0.5f));
shaderProgram.SetUniform3f("viewPos", camera.Position);
ourModel.Draw(&shaderProgram);
// grass
grassShaderProgram.Bind();
grassShaderProgram.SetUniformMat4f("projection", projection);
grassShaderProgram.SetUniformMat4f("view", view);
glBindVertexArray(transparentVAO);
grass.Bind();
for (unsigned int i = 0; i < vegetation.size(); i++)
{
glm::mat4 grassModel(1.0);
grassModel = glm::translate(grassModel, vegetation[i]);
grassShaderProgram.SetUniformMat4f("model", grassModel);
glDrawArrays(GL_TRIANGLES, 0, 6);
}
glBindVertexArray(0);
// 2. Show a simple window that we create ourselves.
// We use a Begin/End pair to created a named window.
{
ImGui::Begin("Application");
ImGui::SliderFloat3("lightPos", &lightPos.x, -2.0f, 2.0f);
ImGui::SliderFloat("rotation", &rotation, -180.0f, 180.0f);
ImGui::Text("Application average %.3f ms/frame (%.1f FPS)", 1000.0f / ImGui::GetIO().Framerate, ImGui::GetIO().Framerate);
ImGui::End();
}
// Rendering
ImGui::Render();
ImGui_ImplOpenGL3_RenderDrawData(ImGui::GetDrawData());
glfwSwapBuffers(window);
glfwPollEvents();
}
glDeleteVertexArrays(1, &skyboxVAO);
glDeleteBuffers(1, &skyboxVBO);
glDeleteVertexArrays(1, &transparentVAO);
glDeleteBuffers(1, &transparentVBO);
}
// Cleanup
ImGui_ImplOpenGL3_Shutdown();
ImGui_ImplGlfw_Shutdown();
ImGui::DestroyContext();
glfwTerminate();
return 0;
}
// process all input: query GLFW whether relevant keys are pressed/released this frame and react accordingly
// ---------------------------------------------------------------------------------------------------------
void processInput(GLFWwindow *window)
{
if (glfwGetKey(window, GLFW_KEY_ESCAPE) == GLFW_PRESS)
glfwSetWindowShouldClose(window, true);
if (glfwGetKey(window, GLFW_KEY_W) == GLFW_PRESS)
camera.ProcessKeyboard(FORWARD, deltaTime);
if (glfwGetKey(window, GLFW_KEY_S) == GLFW_PRESS)
camera.ProcessKeyboard(BACKWARD, deltaTime);
if (glfwGetKey(window, GLFW_KEY_A) == GLFW_PRESS)
camera.ProcessKeyboard(RIGHT, deltaTime);
if (glfwGetKey(window, GLFW_KEY_D) == GLFW_PRESS)
camera.ProcessKeyboard(LEFT, deltaTime);
}
void glfwFramebufferSizeCallback(GLFWwindow *window, int width, int height)
{
glViewport(0, 0, width, height);
}
// glfw: whenever the mouse moves, this callback is called
// -------------------------------------------------------
void mouse_callback(GLFWwindow* window, double xpos, double ypos)
{
if (firstMouse)
{
lastX = xpos;
lastY = ypos;
firstMouse = false;
}
float xoffset = xpos - lastX;
float yoffset = lastY - ypos; // reversed since y-coordinates go from bottom to top
lastX = xpos;
lastY = ypos;
camera.ProcessMouseMovement(xoffset, yoffset);
}
// glfw: whenever the mouse scroll wheel scrolls, this callback is called
// ----------------------------------------------------------------------
void scroll_callback(GLFWwindow* window, double xoffset, double yoffset)
{
camera.ProcessMouseScroll(yoffset);
}
<file_sep>/LeranOpenGL/OpenGLAdvanced/include/TextureCube.h
#pragma once
#include <vector>
class TextureCube
{
public:
TextureCube(const std::vector<std::string> faces);
~TextureCube();
void Bind(unsigned int slot = 0) const;
void Unbind();
private:
unsigned int m_RendererID;
};<file_sep>/LeranOpenGL/OpenGLAdvanced/src/TextureCube.cpp
#include "TextureCube.h"
#include <iostream>
#include <string>
#include "stb_image/stb_image.h"
#include "GL/glew.h"
#include "GLDebug.h"
TextureCube::TextureCube(const std::vector<std::string> faces)
{
glGenTextures(1, &m_RendererID);
glBindTexture(GL_TEXTURE_CUBE_MAP, m_RendererID);
stbi_set_flip_vertically_on_load(0);
int width, height, nrChannels;
for (unsigned int i = 0; i < faces.size(); i++)
{
unsigned char *data = stbi_load(faces[i].c_str(), &width, &height, &nrChannels, 0);
if (data)
{
glTexImage2D(GL_TEXTURE_CUBE_MAP_POSITIVE_X + i, 0, GL_RGB, width, height, 0, GL_RGB, GL_UNSIGNED_BYTE, data);
stbi_image_free(data);
}
else {
std::cout << "Cubemap texture failed to load at path: " << faces[i] << std::endl;
stbi_image_free(data);
}
}
glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE);
glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE);
glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_WRAP_R, GL_CLAMP_TO_EDGE);
}
TextureCube::~TextureCube()
{
//glDeleteTextures(1, &m_RendererID);
}
void TextureCube::Bind(unsigned int slot /* = 0*/) const
{
glActiveTexture(GL_TEXTURE0 + slot);
glBindTexture(GL_TEXTURE_CUBE_MAP, m_RendererID);
}
void TextureCube::Unbind()
{
}
<file_sep>/README.md
# LeranOpenGL
基于 glfw 和 glew 编写 OpenGL
<file_sep>/LeranOpenGL/OpenGLAdvanced/imgui.ini
[Window][Debug##Default]
Pos=60,60
Size=400,400
Collapsed=0
[Window][Application]
Pos=61,27
Size=746,100
Collapsed=0
<file_sep>/LeranOpenGL/OpenGLAdvanced/include/Model.h
#pragma once
#include <iostream>
#include <vector>
#include <assimp/Importer.hpp>
#include <assimp/scene.h>
#include <assimp/postprocess.h>
#include "Mesh.h"
class Model
{
public:
/*函数*/
Model(const std::string &path, bool gamma = false);
~Model();
void Draw(ShaderProgram *shaderProgram) const;
private:
/*模型数据*/
std::vector<Mesh> meshs;
std::string directory;
std::vector<Texture> textures_loaded;
bool gammaCorrection;
/*函数*/
void loadModel(const std::string &path);
void processNode(aiNode *node, const aiScene *scene);
Mesh processMesh(aiMesh *mesh, const aiScene *scene);
std::vector<Texture> loadMaterialTextures(aiMaterial *material, aiTextureType type, std::string typeName);
unsigned int TextureFromFile(const char *path, const std::string diectory, bool gamma = false);
};<file_sep>/LeranOpenGL/OpenGLAdvanced/src/Texture2D.cpp
#include "Texture2D.h"
#include "stb_image/stb_image.h"
#include "GL/glew.h"
#include "GLDebug.h"
Texture2D::Texture2D(const std::string path)
:m_RendererID(0), m_FilePath(path), m_LocalBuffer(NULL),
m_Width(0), m_Height(0), m_BPP(0)
{
stbi_set_flip_vertically_on_load(0);
m_LocalBuffer = stbi_load(path.c_str(), &m_Width, &m_Height, &m_BPP, 4);
GLCall(glGenTextures(1, &m_RendererID));
GLCall(glBindTexture(GL_TEXTURE_2D, m_RendererID));
GLCall(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR));
GLCall(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR));
//GLCall(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE));
//GLCall(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE));
GLCall(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT));
GLCall(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT));
GLCall(glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA8, m_Width, m_Height, 0, GL_RGBA, GL_UNSIGNED_BYTE, m_LocalBuffer));
GLCall(glGenerateMipmap(GL_TEXTURE_2D));
if (m_LocalBuffer)
{
stbi_image_free(m_LocalBuffer);
}
}
Texture2D::~Texture2D()
{
GLCall(glDeleteTextures(1, &m_RendererID));
}
void Texture2D::Bind(unsigned int slot /* = 0*/) const
{
GLCall(glActiveTexture(GL_TEXTURE0 + slot));
GLCall(glBindTexture(GL_TEXTURE_2D, m_RendererID));
}
void Texture2D::Unbind() const
{
}
<file_sep>/LeranOpenGL/OpenGLAdvanced/include/Mesh.h
#pragma once
#include <iostream>
#include <vector>
#include "glm/glm.hpp"
#include "assimp/types.h"
class ShaderProgram;
struct Vertex
{
glm::vec3 Position;
glm::vec3 Normal;
glm::vec2 TexCoords;
};
struct Texture
{
unsigned int id;
std::string type;
aiString path; // 我们储存纹理的路径用于与其它纹理进行比较
};
class Mesh
{
public:
/*函数*/
Mesh(std::vector<Vertex> _vertices, std::vector<unsigned int> _indices, std::vector<Texture> _textures);
~Mesh();
void Draw(ShaderProgram *shaderProgram) const;
inline unsigned int GetVAO() const { return VAO; }
inline unsigned int GetVBO() const { return VBO; }
inline unsigned int GetEBO() const { return EBO; }
private:
/*网格数据*/
std::vector<Vertex> vertices;
std::vector<unsigned int> indices;
std::vector<Texture> textures;
/*渲染数据*/
unsigned int VAO;
unsigned int VBO;
unsigned int EBO;
/*函数*/
void SetupMesh();
};
| 9ecb37bf060c3bb5bfbd86a3064fcc226b51021e | [
"Markdown",
"C++",
"INI"
] | 10 | C++ | SunHailang/LeranOpenGL | a7d923626fe30ab62ca202c8118bc5804aaa1086 | 8ad24695e43ae5108fb41a11a193d550cb3e2224 | |
refs/heads/master | <repo_name>Tenchberry/YMF_scripts<file_sep>/animate_MD.py
#Animated plot of the system state on the potential energy over the simulation time
from triplepotentialMD import *
import matplotlib
matplotlib.rcParams['text.usetex'] = False
import matplotlib.animation as animation
from matplotlib.animation import ImageMagickFileWriter
writer = ImageMagickFileWriter()
#-----------------------------------------------------------------------------------
#Setup of MD_TriplePotential class instance
x_0 = 1.9
v_0 = 2.2 #2.2 #Saving initial velocities and coordinates to position and velocity vectors
MDSteps = int(500)
dt = 0.01
Test_VV3W = MD_TriplePotential(x_0, v_0, MDSteps, dt, 100)
#Running simulation and generating output arrays
for i in range(1, MDSteps):
Test_VV3W.step(i)
x_trj = Test_VV3W.get_xarr
epot = Test_VV3W.get_epot
#-----------------------------------------------------------------------------------
# set up figure and animation
x = np.linspace(0.125, 3.5, 1000)
fig = plt.figure(figsize=(12,6))
ax = fig.add_subplot(111, autoscale_on=False,
xlim=(0.125, 3.5), ylim=(-0.2, 8.0))
ax.grid()
#Build the triple well potential
A = [1.5, 1.8, 2]
a = 1.7
q = (2*math.pi)/a
potential = [(A[0]*math.cos(q*x[i]) + A[1]*math.cos(1.5*q*x[i]) + A[2]*math.sin(1.5*q*x[i]) + 4)
for i in np.arange(0, len(x))]
minval = [-0.2 for i in np.arange(0, len(x))]
#ax.set_title(r'Test MD', fontsize=16, weight='bold')
ax.plot(x, potential, linestyle="-", color="purple")
ax.plot(x, minval, linestyle="-", color="black")
ax.fill_between(x, minval, potential, facecolor="black")
ax.set_xlabel(r'CV Value', style='oblique', fontsize=20)
ax.set_ylabel(r'Free Energy (kJ/mol)', style='oblique', fontsize=20)
#matplotlib.rcParams['text.usetex']=False
point, = ax.plot([], [], "o", color="red", markersize=12)
time_text = ax.text(0.02, 0.95, '', transform=ax.transAxes)
energy_text = ax.text(0.02, 0.90, '', transform=ax.transAxes)
def init():
"""initialize animation"""
matplotlib.rcParams['text.usetex'] = False
point.set_data([], [])
time_text.set_text('')
energy_text.set_text('')
return point, time_text, energy_text
def animate(i):
"""perform animation step"""
global x_trj, epot
new_data = (x_trj[i], epot[i]+0.15)
print(new_data)
point.set_data(*new_data)
#time_text.set_text(r'X = %.1f' % new_data[0])
#energy_text.set_text(r'Energy = %.3f kJ/mol' % epot[i])
return point, time_text, energy_text
# choose the interval based on dt and the time to animate one step
interval = 10
ani = animation.FuncAnimation(fig, animate, frames=400,
interval=interval, blit=True, init_func=init)
#Save the animation to a gif
ani.save('MDhot_test.gif', writer=writer)
fig.tight_layout()
plt.show()
<file_sep>/README.md
# YMF_scripts
A library of all the scripts used to generate animations for the YMF 2019 Presentation - "Towards more selective enhanced sampling of biomolecular dynamics"
<file_sep>/animate_plots.py
import mdtraj
import matplotlib.pyplot as plt
from matplotlib import rc
import numpy as np
import seaborn as sns
from scipy.interpolate import griddata
from scipy import math
import matplotlib.animation as animation
from matplotlib.animation import ImageMagickFileWriter
writer = ImageMagickFileWriter()
sns.set_style("dark")
maxr = 200
colvar = np.loadtxt("COLVAR_HMC", skiprows=31800, max_rows=maxr)
colvar_short = np.loadtxt("COLVAR_MD",skiprows=24222, max_rows=maxr)
#colvar_long = np.loadtxt("COLVAR_longTDHMC", max_rows=maxr)
fig = plt.figure(figsize=(12,6))
surface_file = np.loadtxt("fes_39.dat", usecols=(0,1,2))
phi, psi = np.meshgrid(surface_file[:,0], surface_file[:,1])
pos = np.array([surface_file[:,0], surface_file[:,1]]).T
Z = griddata(pos, surface_file[:,2], (phi, psi), method='cubic')
levels=[0, 10, 20, 30, 40, 50, 60, 70, 80]
ax = fig.add_subplot(121)
ax.set_title(r'HMC - 10fs step', fontsize=20)
HMC_plot, = ax.plot([], [], "o-", lw=0.2, color="black")
ax.contour(phi, psi, Z, levels, cmap='rainbow')
ax.contourf(phi, psi, Z, levels, cmap='rainbow')
ax.set_xlim(-math.pi, math.pi)
ax.set_ylim(-math.pi, math.pi)
ax.set_xlabel(r'$\phi$', fontsize=20)
ax.set_ylabel(r'$\psi$', fontsize=20)
ax.grid('off')
ax_md = fig.add_subplot(122)
ax_md.set_title(r'MD - Velocity Verlet (1fs)', fontsize=20)
MD_plot, = ax_md.plot([], [], "o-", lw=0.2, color="blue")
ax_md.contour(phi, psi, Z, levels, cmap='rainbow')
ax_md.contourf(phi, psi, Z, levels, cmap='rainbow')
ax_md.set_xlabel(r'$\phi$', fontsize=20)
ax_md.set_ylabel(r'$\psi$', fontsize=20)
ax_md.set_xlim(-math.pi, math.pi)
ax_md.set_ylim(-math.pi, math.pi)
ax_md.grid('off')
#ax_tmd = fig.add_subplot(133)
#ax_tmd.set_title(r'TDHMC - 700fs step', fontsize=20)
#TMD_plot, = ax_tmd.plot([], [], "o-", lw=0.2, color="blue")
#ax_tmd.contour(phi, psi, Z, levels, cmap='rainbow')
#ax_tmd.contourf(phi, psi, Z, levels, cmap='rainbow')
#ax_tmd.set_xlabel(r'$\phi$', fontsize=20)
#ax_tmd.set_ylabel(r'$\psi$', fontsize=20)
#ax_tmd.set_xlim(-math.pi, math.pi)
#ax_tmd.set_ylim(-math.pi, math.pi)
#ax_tmd.grid('off')
def init():
"""initialize animation"""
HMC_plot.set_data([], [])
MD_plot.set_data([], [])
#TMD_plot.set_data([], [])
return HMC_plot, MD_plot #, TMD_plot
def animate(i):
"""perform animation step"""
global colvar, colvar_short # colvar_long
HMC_data = (colvar[:i,1], colvar[:i,2])
MD_data = (colvar_short[:i,1], colvar_short[:i,2])
#TMD_data = (colvar_long[:i,1], colvar_long[:i,2])
print(colvar[i,0], colvar[i,1], colvar[i,2])
HMC_plot.set_data(HMC_data)
MD_plot.set_data(MD_data)
#TMD_plot.set_data(TMD_data)
return HMC_plot , MD_plot #, TMD_plot
# choose the interval based on dt and the time to animate one step
interval = 10
ani = animation.FuncAnimation(fig, animate, frames=maxr,
interval=interval, blit=True, init_func=init)
#Save the animation to a gif
ani.save('HMCvsMD.gif', writer=writer)
fig.tight_layout()
plt.show()
<file_sep>/triplepotentialMD.py
from typing import Optional, Any
import numpy as np
import matplotlib
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
from scipy import math
import scipy.stats as stats
# matplotlib.rcParams['text.usetex'] = True
import seaborn as sns
sns.set()
class MD_TriplePotential:
"""
1D MD simulation over a triple well potential using the symplectic
Velocity Verlet integrator
Potential - V(x) 4 + 1.5cos(2pix/1.7) + 1.8cos(3pix/1.7) + 2sin(3pix/1.7)
Force - F(x) = -dV(x)/dx = (6pi/17)(5sin(2pix/1.7) + 9sin(3pix/1.7) - 10cos(3pix/1.7))
Starting coordinate fixed at 1.9, but is tunable
"""
def __init__(self, x_i, v_i, n_steps, dt, printfreq=100, mass=1.0):
# Initialising arrays for x, v and energies
x_trj = np.zeros(n_steps, dtype=np.float64)
v_trj = np.zeros(n_steps, dtype=np.float64)
x_trj[0] = x_i
v_trj[0] = v_i
Epot = np.zeros(n_steps, dtype=np.float64)
Ekin = np.zeros(n_steps, dtype=np.float64)
Etot = np.zeros(n_steps, dtype=np.float64)
self.x_arr = x_trj
self.v_arr = v_trj
self.epot = Epot
self.ekin = Ekin
self.etot = Etot
self.dt = dt
self.mass = mass
self.freq = printfreq
self.intervals = (0.125, 3.5)
# Setting functions for calculation of forces, potential energy and kinetic energy
def calc_Force(self, x, a=1.7):
q = ((2 * math.pi) / a)
return (6 * math.pi / 17) * (5.0 * math.sin(q * x) + 9.0 * math.sin(1.5 * q * x) - 10.0 * math.cos(1.5 * q * x))
def calc_TriplePotential(self, x, a=1.7):
q = ((2 * math.pi) / a)
return 4.0 + 1.5 * math.cos(q * x) + 1.8 * math.cos(1.5 * q * x) + 2.0 * math.sin(1.5 * q * x)
def calc_KineticEnergy(self, v):
return 0.5 * self.mass * (v ** 2)
# Functions for performing Velocity Verlet integrations of positions and coordinates
def VelocityVerletUpdate_position(self, x, v, stepf=1.0):
return x + v * self.dt * stepf
def VelocityVerletUpdate_velocity(self, v, F, stepf=1.0):
return v + (0.5 * self.dt * stepf) * F
# Function for Velocity Verlet MD update
def step(self, step):
x = self.x_arr[step - 1]
v = self.v_arr[step - 1]
self.epot[step - 1] = self.calc_TriplePotential(x)
self.ekin[step - 1] = self.calc_KineticEnergy(v)
self.etot[step - 1] = self.ekin[step - 1] + self.epot[step - 1]
if (step % self.freq == 0):
print("Step %d - X = %f \n Potential = %f kJ/mol, Kinetic = %f kJ/mol, TOTAL = %f kJ/mol"
% (step, self.x_arr[step - 1], self.epot[step - 1], self.ekin[step - 1], self.etot[step - 1]))
f = self.calc_Force(x)
v = self.VelocityVerletUpdate_velocity(v, f, stepf=0.5)
x = self.VelocityVerletUpdate_position(x, v, stepf=1.0)
f = self.calc_Force(x)
v = self.VelocityVerletUpdate_velocity(v, f, stepf=0.5)
if (x >= self.intervals[1]) or (x<= self.intervals[0]):
print("System is out of bounds - reverting to previous position")
v = self.VelocityVerletUpdate_velocity(v, f, stepf=-2.5)
x = self.VelocityVerletUpdate_position(x, v, stepf=-5.0)
v = self.VelocityVerletUpdate_velocity(v, f, stepf=-2.5)
self.x_arr[step] = x
self.v_arr[step] = v
@property
def get_outputs(self):
return self.x_arr, self.v_arr, self.epot, self.ekin, self.etot
@property
def get_xarr(self):
return self.x_arr
@property
def get_varr(self):
return self.v_arr
@property
def get_epot(self):
return self.epot
@property
def get_ekin(self):
return self.ekin
@property
def get_etot(self):
return self.etot
class MetaD_TriplePotential(MD_TriplePotential):
"""
1D metadynamics (MetaD) simulation over a triple well potential using the symplectic
Velocity Verlet integrator
Collective Variable (CV) for the MetaD bias is the X value for the 1D particle
Potential - V(x) 4 + 1.5cos(2pix/1.7) + 1.8cos(3pix/1.7) + 2sin(3pix/1.7)
Force - F(x) = -dV(x)/dx = (6pi/17)(5sin(2pix/1.7) + 9sin(3pix/1.7) - 10cos(3pix/1.7))
Starting coordinate fixed at 1.9, but is tunable
"""
def __init__(self, x_i, v_i, Gauss_width, Gauss_height,
n_steps, dt, printfreq=100, mass=1.0):
super().__init__(x_i, v_i, n_steps, dt, printfreq, mass)
#Number of points in biasgrid over which the Gaussian biases are plotted - Currently hard coded
self.gausspoints = 100
#MetaD Gaussian kernel parameters - Height is the maximum frequency value of the kernel
# and width defines the kernel standard deviation
self.gwidth = Gauss_width
self.gheight = Gauss_height
#Initialising grid of the explorable 1D space (CV space grid) and explorable unbiased potential
#X_grid = np.linspace(0.125, 3.5, 100000)
# A = [1.5, 1.8, 2]
# a = 1.7
# q = (2 * math.pi) / a
# V_unbiased = [(A[0] * math.cos(q * X_grid[i]) + A[1] * math.cos(1.5 * q * X_grid[i]) + A[2] * math.sin(1.5 * q * X_grid[i]) + 4)
# for i in np.arange(0, len(X_grid))]
#
# V_grid = np.array([X_grid, V_unbiased]).T
#
# self.xgrid = X_grid
# self.Vg = V_grid #Will have Gaussians added to it at each deposition step
#Initialise dictionary of Gaussian kernels
# - Keys - Center of the kernel
# - Values - the density values of the kernel
#arraylength = int(n_steps/self.freq)
bias_dict = {}
self.bias = bias_dict
def __calc_biasGaussian(self, x):
"""
Constructing the additive Gaussian bias using input width and height
N.B. Only for listing the accumulated Gaussians into the bias grid -
The bias potential is implemented into the dynamics using the calc_biasForce() and
add_biasPotential() attributes
:param x:
:return gaussian:
"""
gauss_range = np.linspace(x - 3 * self.gwidth, x + 3 * self.gwidth, self.gausspoints)
gauss_ = self.gheight * stats.norm.pdf(gauss_range, x, self.gwidth)
gaussian = np.array([gauss_range, gauss_]).T
return gaussian
def __add_biasPotential(self, epot):
"""
Calculating the effective potential at t = tau_G, by adding the computed Gaussian
kernel to the grid potential
:param x: The center of the Gaussian kernel
:param epot: The current potential energy
:param gaussian: The Gaussian kernel to be added
:return: Vg - The current effective potential
"""
#First searching for xspace in which to deposit the Gaussian
#for i in range(0, len(self.Vg[:,0])):
# if Vg
epot_ebias = epot + self.gheight
return epot_ebias
def __calc_biasForce(self, x):
"""
Gaussian bias force is calculated as the derivative of the gaussian with respect to the CV x
Note - Implements practically the biasforce as the maximum value of the derivative -dVg/dx
:param x:
:return gauss_force:
"""
gauss_range = np.linspace(x - 3 * self.gwidth, x + 3 * self.gwidth, 100)
der_coeff = np.array([((gauss_range[i] - x)/(self.gwidth**2)) for i in range(0, len(gauss_range))]
, dtype=np.float64)
gaussforce = np.array(self.gheight * der_coeff * stats.norm.pdf(gauss_range, x, self.gwidth))
gauss_force = np.max(gaussforce)
return gauss_force
def step(self, step):
x = self.x_arr[step - 1]
v = self.v_arr[step - 1]
self.epot[step - 1] = self.calc_TriplePotential(x)
self.ekin[step - 1] = self.calc_KineticEnergy(v)
self.etot[step - 1] = self.ekin[step - 1] + self.epot[step - 1]
if (step % self.freq == 0):
# Bias generation - Gaussian bias potential is constructed at x(tau_G)
self.bias[step] = self.__calc_biasGaussian(x)
self.epot[step - 1] = self.__add_biasPotential(self.epot[step - 1])
print("Step %d - X = %f \n Potential = %f kJ/mol, Kinetic = %f kJ/mol, TOTAL = %f kJ/mol"
% (step, self.x_arr[step - 1], self.epot[step - 1], self.ekin[step - 1], self.etot[step - 1]))
f = self.calc_Force(x) + self.__calc_biasForce(x)
v = self.VelocityVerletUpdate_velocity(v, f, stepf=0.5)
x = self.VelocityVerletUpdate_position(x, v, stepf=1.0)
f = self.calc_Force(x) + self.__calc_biasForce(x)
v = self.VelocityVerletUpdate_velocity(v, f, stepf=0.5)
else:
f = self.calc_Force(x)
v = self.VelocityVerletUpdate_velocity(v, f, stepf=0.5)
x = self.VelocityVerletUpdate_position(x, v, stepf=1.0)
f = self.calc_Force(x)
v = self.VelocityVerletUpdate_velocity(v, f, stepf=0.5)
self.x_arr[step] = x
self.v_arr[step] = v
@property
def get_bias(self):
return self.bias
# Deleting (Calling destructor)
def __del__(self):
print('Destructor called, Simulation terminated abnormally')
| ad13f69c18cc61e192b2f9ed851ffa3aa519b9ba | [
"Markdown",
"Python"
] | 4 | Python | Tenchberry/YMF_scripts | c5334e0edf2374c3af6fc4f8e0513860c70a93ac | 62b16885428ff936664c9344171dab2c4e19db5a | |
refs/heads/master | <repo_name>by-HuHu/RTU-485<file_sep>/reading_registers_from_iEM3155.py
#!/usr/bin/env
# The script reads registers from the iEM3155 by using the Modbus RTU protocol
# Register Address has to be the Register Address number minus 1
import serial
import time
import datetime
import minimalmodbus
minimalmodbus.CLOSE_PORT_AFTER_EACH_CALL = True
minimalmodbus.HANDLE_LOCAL_ECHO = False
instr = minimalmodbus.Instrument('COM16', 1 , mode = 'rtu')
# Arguments: Port name (str), Slave adress (decimal), mode (RTU or ASCII)
# Port name: The serial port name, for example /dev/ttyUSB0 (Linux), /dev/tty.usbserial (OS X) or COM4 (Windows).
instr.serial.baudrate = 19200
instr.serial.bytesize = 8
instr.serial.stopbits = 1
instr.serial.parity = serial.PARITY_NONE
instr.serial.timeout = 1
instr.debug = False
def convert_64bit(register):
""" Converts the varaiables from Int16 to Int64 """
real_value = (register[0]*(2^48) + register[1]*(2^32) + register[2]*(2^16) + register[3]) / 1000.
return (real_value)
def collect_data():
""" Collects the varaiable from the instrument, it reads four Int16 and then it converts them into one Int64 """
try:
register_3204 = instr.read_registers(3203,4,3) #Total Active Energy Import -kWh-
register_3208 = instr.read_registers(3207,4,3) #Total Active Energy Export -kWh-
register_3220 = instr.read_registers(3219,4,3) #Total reactive Energy Import -kVARh-
register_3224 = instr.read_registers(3223,4,3) #Total reactive Energy Export -kVARh-
totalEaImport = convert_64bit(register_3204)
totalEaExport = convert_64bit(register_3208)
totalErImport = convert_64bit(register_3220)
totalErExport = convert_64bit(register_3224)
data = [totalEaImport, totalEaExport, totalErImport, totalErExport]
except IOError:
print("Failed to read from instrument")
return (data)
while True:
dt = datetime.datetime.now().strftime('%Y/%m/%d %H:%M:%S')
data = collect_data()
data_ls = [dt, str(data[0]), str(data[1]), str(data[2]), str(data[3])]
print(data)
data_str = ",".join(data_ls)
print(data_str)
time.sleep(10)
<file_sep>/README.md
# RTU-485 Schneider iEM3155
RTU-485 communication protocol with Python for reading registers from the Schneider iEM3155.
| c86cab386d52a7c1f2a89611f553405808a93969 | [
"Markdown",
"Python"
] | 2 | Python | by-HuHu/RTU-485 | e9a4f555638eac5ae47ec065e3be19930c8b0a0e | 0802f48d312df608335e7098d59517b9831727cb | |
refs/heads/master | <file_sep>#include "solarsystem.hpp"
#include <cstdlib>
#define REST 700
#define REST_Z (REST)
#define REST_Y (-REST)//初始视角在(x,-x,x)处
void SolarSystem::onDisplay(){
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glClearColor(.7f,.7f,.7f,.1f);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(75.0f,1.0f,1.0f,40000000);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
gluLookAt(viewX,viewY,viewZ,centerX,centerY,centerZ,upX,upY,upZ);
glEnable(GL_LIGHT0);
glEnable(GL_LIGHTING);
glEnable(GL_DEPTH_TEST);
for(int i=0;i<STARS_NUM;i++)
stars[i]->draw();
glutSwapBuffers();
}
#define TIMEPAST 1 //假设每次更新都经历了一天
void SolarSystem::onUpdate(){
for(int i=0;i<STARS_NUM;i++)
stars[i] ->update(TIMEPAST); //更新星球位置
this ->onDisplay();//刷新显示
}
#define OFFSET 20
void SolarSystem::onKeyboard(unsigned char key,int x,int y){
switch (key) {
case 'w': viewY += OFFSET; break;
case 's': viewZ += OFFSET; break;
case 'S': viewZ -= OFFSET; break;
case 'a': viewX -= OFFSET; break;
case 'd': viewX += OFFSET; break;
case 'x': viewY -= OFFSET; break;
case 'r':
viewX = 0;viewY = REST_Y;viewZ = REST_Z;
centerX = centerY = centerZ = 0;
upX = upY = 0;upZ = 1;
break;
case 27:exit(0);break;
default:break;
}
}
#define SUN_RADIUS 48.74
#define MER_RADIUS 7.32
#define VEN_RADIUS 18.15
#define EAR_RADIUS 19.13
#define MOO_RADIUS 6.15
#define MAR_RADIUS 10.19
#define JUP_RADIUS 42.90
#define SAT_RADIUS 36.16
#define URA_RADIUS 25.56
#define NEP_RADIUS 24.78
#define MER_DIS 62.06
#define VEN_DIS 115.56
#define EAR_DIS 168.00
#define MOO_DIS 26.01
#define MAR_DIS 228.00
#define JUP_DIS 333.40
#define SAT_DIS 428.10
#define URA_DIS 848.00
#define NEP_DIS 949.10
#define MER_SPEED 87.0
#define VEN_SPEED 225.0
#define EAR_SPEED 365.0
#define MOO_SPEED 30.0
#define MAR_SPEED 687.0
#define JUP_SPEED 1298.4
#define SAT_SPEED 3225.6
#define URA_SPEED 3066.4
#define NEP_SPEED 6014.8
#define SELFROTATE 3
enum STARS {Sun, Mercury, Venus, Earth, Moon,
Mars, Jupiter, Saturn, Uranus, Neptune};
#define SET_VALUE_3(name,value0,value1,value2) \
((name)[0] = (value0),(name)[1] = (value1),(name)[2] = (value2))
SolarSystem::SolarSystem(){
viewX = 0;
viewY = REST_Y;
viewZ = REST_Z;
centerX = centerY = centerZ = 0;
upX = upY = 0;
upZ = 1;
GLfloat rgbaColor[3] = {1,0,0};
stars[Sun] = new LightPlanet(SUN_RADIUS,0,0,SELFROTATE,0,rgbaColor);
SET_VALUE_3(rgbaColor, .1, .7, 0);
stars[Venus] = new Planet(VEN_RADIUS,VEN_DIS,VEN_SPEED,SELFROTATE,stars[Sun],rgbaColor);
SET_VALUE_3(rgbaColor, 0, 1, 0);
stars[Earth] = new Planet(EAR_RADIUS,EAR_DIS,EAR_SPEED,SELFROTATE,stars[Sun],rgbaColor);
SET_VALUE_3(rgbaColor, 1, 1, 0);
stars[Moon] = new Planet(MOO_RADIUS,MOO_DIS,MOO_SPEED,SELFROTATE,stars[Sun],rgbaColor);
SET_VALUE_3(rgbaColor, 1, .5, .5);
stars[Mars] = new Planet(MAR_RADIUS,MAR_DIS,MAR_SPEED,SELFROTATE,stars[Sun],rgbaColor);
SET_VALUE_3(rgbaColor, 1, 1, .5);
stars[Jupiter] = new Planet(JUP_RADIUS,JUP_DIS,JUP_SPEED,SELFROTATE,stars[Sun],rgbaColor);
SET_VALUE_3(rgbaColor, .5, 1, .5);
stars[Saturn] = new Planet(SAT_RADIUS,SAT_DIS,SAT_SPEED,SELFROTATE,stars[Sun],rgbaColor);
SET_VALUE_3(rgbaColor, .4, .4, .4);
stars[Uranus] = new Planet(URA_RADIUS,URA_DIS,URA_SPEED,SELFROTATE,stars[Sun],rgbaColor);
SET_VALUE_3(rgbaColor, .5, .5, 1);
stars[Neptune] = new Planet(NEP_RADIUS,NEP_DIS,NEP_SPEED,SELFROTATE,stars[Sun],rgbaColor);
}
SolarSystem::~SolarSystem(){
for(int i = 0; i < STARS_NUM; i++)
delete stars[i];
}
<file_sep>#ifndef stars_hpp
#define stars_hpp
#ifdef __APPLE__
#include <GLUT/glut.h>
#else
#include <GL/glut.h>
#endif
class Star{
public:
GLfloat radius;//运行半径
GLfloat speed,selfSpeed;//公转 自转速度
GLfloat distance;//星球中心与父节点星球中心的距离
GLfloat rgbaColor[4];//星球的颜色
Star* parentStar;//父节点颜色
Star(GLfloat radius,GLfloat distance,GLfloat speed,//构造函数
GLfloat selfSpeed,Star* parent);
void drawStar();//对一般的星球的移动 旋转等活动进行绘制
virtual void draw(){
drawStar();//提供默认实现 负责调用drawStar()
}
virtual void update(long timeSpan);//参数为每次刷新画面时的时间跨度
protected:
GLfloat alphaSelf, alpha;
};//当前的自转角度 公转角度
class Planet:public Star{
public:
Planet(GLfloat radius,GLfloat distance,
GLfloat speed,GLfloat selfSpeed,
Star* parent,GLfloat rgbaColor[3]);
void drawPlanet();//增加对具备自身材质的行星绘制材质
virtual void draw(){
drawPlanet(); drawStar();}//继续向其子类开放重写功能
};
class LightPlanet:public Planet{
public:
LightPlanet(GLfloat Radius,GLfloat Distance,
GLfloat Speed,GLfloat SelfSpeed,
Star* Parent,GLfloat rgbaColor[]);
void drawLight();//增加对提供光源的恒星绘制光照
virtual void draw(){
drawLight();drawPlanet();drawStar();
}
};
#endif
<file_sep># Shiyanlou
实验楼C++学习项目
<file_sep>#include "stars.hpp"
#include <cmath>
#define PI 3.141592635
Star::Star(GLfloat radius,GLfloat distance,GLfloat speed,GLfloat selfSpeed,Star* parent){
this->radius = radius;
this->selfSpeed = selfSpeed;
this->alphaSelf = this->alpha = 0;
this->distance = distance;
for(int i= 0;i < 4,i++;)
this->rgbaColor[i] = 1.0f;
this->parentStar = parent;
if(speed > 0)
this->speed = 360.0f / speed;
else
this->speed = 0.0f;
}
void Star::drawStar(){
glEnable(GL_LINE_SMOOTH);
glEnable(GL_BLEND);
int n = 1440;
glPushMatrix();//保存OpenGL当前的工作环境
{
// 公转
// 如果是行星,且距离不为0,那么 且向原点平移一个半径
// 这部分用于处理卫星
if (parentStar != 0 && parentStar -> distance >0){
//将绘制的图形沿Z轴旋转alpha
glRotatef(parentStar -> alpha,0,0,1);
//X轴方向上平移distance,y,z 方向不变
glTranslatef(parentStar ->distance,0.0,0.0);
}
//绘制运行轨道
glBegin(GL_LINES);
for(int i=0;i<n;++i)
glVertex2f(distance * cos(2*PI*i/n),distance*sin(2*PI*i/n));
glEnd();
glRotatef(alpha,0,0,1);
glTranslatef(distance,0.0,0.0);
glRotatef(alphaSelf,0,0,1);
//绘制行星颜色
glColor3f(rgbaColor[0],rgbaColor[1],rgbaColor[2]);
glutSolidSphere(radius,40,32);
}
glPopMatrix();//恢复当前矩阵环境
}
void Star::update(long timeSpan){
alpha += timeSpan * speed;
alphaSelf += selfSpeed;
}
Planet::Planet(GLfloat radius,GLfloat distance,GLfloat speed,
GLfloat selfSpeed,Star* parent,GLfloat rgbaColor[3]):
Star(radius,distance,speed,selfSpeed,parent){
rgbaColor[0] = rgbaColor [0];
rgbaColor[1] = rgbaColor [1];
rgbaColor[2] = rgbaColor [2];
rgbaColor[3] = 1.0f;
}
//不发光的星球
void Planet::drawPlanet(){
GLfloat mat_ambient[] = {0.0f,0.0f,0.5f,1.0f};
GLfloat mat_diffuse[] = {0.0f,0.0f,0.5f,1.0f};
GLfloat mat_specular[] = {0.0f,0.0f,1.0f,1.0f};
GLfloat mat_emission[] = {rgbaColor[0],rgbaColor[1],rgbaColor[2],rgbaColor[3]};
GLfloat mat_shininess = 90.0f;
glMaterialfv(GL_FRONT, GL_AMBIENT, mat_ambient);
glMaterialfv(GL_FRONT,GL_DIFFUSE,mat_diffuse);
glMaterialfv(GL_FRONT,GL_SPECULAR,mat_specular);
glMaterialfv(GL_FRONT,GL_EMISSION,mat_emission);
glMaterialf(GL_FRONT,GL_SHININESS,mat_shininess);
}
LightPlanet::LightPlanet(GLfloat radius,GLfloat distance,GLfloat speed,
GLfloat selfSpeed,Star* parent,GLfloat rgbaColor[3]):
Planet(radius,distance,speed,selfSpeed,parent,rgbaColor){
;
}
void LightPlanet::drawLight(){
GLfloat light_position[] = {0.0f,0.0f,0.0f,1.0f};
GLfloat light_ambient[] = {0.0f,0.0f,0.0f,1.0f};
GLfloat light_diffuse[] = {1.0f,1.0f,1.0f,1.0f};
GLfloat light_specular[] = {1.0f,1.0f,1.0f,1.0f};
glLightfv(GL_LIGHT0,GL_POSITION,light_position);
glLightfv(GL_LIGHT0,GL_AMBIENT,light_ambient);
glLightfv(GL_LIGHT0,GL_DIFFUSE,light_diffuse);
glLightfv(GL_LIGHT0,GL_SPECULAR,light_specular);
}
<file_sep>/*
思路:
1,初始化星球对象
2.初始化OpenGL引擎,实现onDraw和onUpdate;
3.星球应该自己负责处理自己的属性,绕行关系,变换相关绘制,因此在设计星球的类时应该提供一个绘制draw()的方法;
4.星球也应该处理自己自转公转等更新显示的绘制,因此提供更新方法update();
5.在onDraw()中应该调用星球draw()方法;
6.在onUpdate()中调用星球update()方法;
7.在onKeyboard()调整整个太阳系的显示;
*/
#ifdef __APPLE__
#include <GLUT/glut.h>
#else
#include <GL/glut.h>
#endif
#include "solarsystem.hpp"
//创建图形窗口的基本宏
#define WINDOW_X_POS 50
#define WINDOW_Y_POS 50
#define WIDTH 700
#define HEIGHT 700
SolarSystem solarsystem;
//用于注册GLUT的回调
void onDisplay(void){
solarsystem.onDisplay();
}
void onUpdate(void){
solarsystem.onUpdate();
}
void onKeyboard(unsigned char key,int x,int y) {
solarsystem.onKeyboard(key,x,y);
}
int main(int argc,char* argv[]){
glutInit(&argc,argv); //对GLUT进行初始化,并处理所有的命令行参数
glutInitDisplayMode(GLUT_RGBA | GLUT_DOUBLE);//使用RGBA模式韩式索引模式;双缓冲窗口
glutInitWindowPosition(WINDOW_X_POS,WINDOW_Y_POS);//设置窗口被创建时左上角位于屏幕上的位置
glutCreateWindow("My solarsystem");//窗口的标题
glutDisplayFunc(onDisplay);//
glutIdleFunc(onUpdate);
glutKeyboardFunc(onKeyboard);
glutMainLoop();
return 0;
}
<file_sep>#ifndef solarsystem_hpp
#define solarsystem_hpp
#ifdef __APPLE__
#include <GLUT/glut.h>
#else
#include<GL/glut.h>
#endif
#include "stars.hpp"
//#define TIMEPAST 1
//#define SELFROTATE 3
#define STARS_NUM 10
class SolarSystem{
public:
SolarSystem();
~SolarSystem();
void onDisplay();
void onUpdate();
void onKeyboard(unsigned char key,int x,int y);
private:
Star* stars[STARS_NUM];
//定义观察视角的参数
GLdouble viewX, viewY, viewZ;
GLdouble centerX, centerY, centerZ;
GLdouble upX, upY, upZ;
};
#endif
<file_sep>//白色方框
/*#include <GL/glut.h>
void display(){
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_POLYGON);
glVertex2f(-0.5,-0.5);
glVertex2f(-0.5,0.5);
glVertex2f(0.5,0.5);
glVertex2f(0.5,-0.5);
glEnd();
glFlush();
}
int main(int argc,char *argv[]){
glutInit(&argc,argv);
glutCreateWindow("Simple");
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
*/
// 红色网格状茶壶
// 编译命令 gcc -o test test.c -lGL -lGLU -lglut
#include <GL/glut.h>
void init(void)
{
glClearColor(0.0, 0.0, 0.0, 0.0);
glMatrixMode(GL_PROJECTION);
glOrtho(-5, 5, -5, 5, 5, 15);
glMatrixMode(GL_MODELVIEW);
gluLookAt(0, 0, 10, 0, 0, 0, 0, 1, 0);
return;
}
void display(void)
{
glClear(GL_COLOR_BUFFER_BIT);
glColor3f(1.0, 0, 0);
glutWireTeapot(3);
glFlush();
return;
}
int main(int argc, char *argv[])
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_RGB | GLUT_SINGLE);
glutInitWindowPosition(0, 0);
glutInitWindowSize(300, 300);
glutCreateWindow("OpenGL 3D View");
init();
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
| 6b0b916de0d6979c3a2109358e0cb3882aa008d1 | [
"Markdown",
"C",
"C++"
] | 7 | C++ | zh805/Shiyanlou | 0a613c35abe4e88892c8fb5b0a58a28099eff481 | 543fa38a4663d8f3b58456224b9fadf65beb1f67 | |
refs/heads/master | <repo_name>yb1994917/Awesome-XposedWechat<file_sep>/README.md
# 微信Hook工具(适配微信6.7.3)
目前收集的有
- hook微信的日志(便于查看关键调用点)
- 自造微信日志异常栈(便于查看代码调用)
- hook Activity跳转
<file_sep>/app/src/main/java/com/demo/xposed/hook/HookDB.java
/**
* HookDB 2019-05-24
* Copyright (c) 2019 YiYeHuDong Co.ltd. All right reserved.
**/
package com.demo.xposed.hook;
import android.content.ContentValues;
import android.content.Context;
import android.util.Log;
import de.robv.android.xposed.XC_MethodHook;
import de.robv.android.xposed.XposedHelpers;
import de.robv.android.xposed.callbacks.XC_LoadPackage;
/**
*@desc
*@quthor tangminglong
*@since 2019-05-24
*/
public class HookDB {
private static final String TAG = HookDB.class.getSimpleName();
private static HookDB instance;
public static HookDB getInstance() {
if (instance == null){
synchronized (HookDB.class){
if (instance == null){
instance= new HookDB();
}
}
}
return instance;
}
public void hook(XC_LoadPackage.LoadPackageParam loadPackageParam){
try {
Class<?> aClass = loadPackageParam.classLoader.loadClass("com.tencent.wcdb.database.SQLiteDatabase");
XposedHelpers.findAndHookMethod(aClass, "insert", String.class, String.class, ContentValues.class, new XC_MethodHook() {
@Override
protected void afterHookedMethod(MethodHookParam param) throws Throwable {
super.afterHookedMethod(param);
Log.e(TAG,"InsertDBLOG");
Object arg = param.args[10];
}
});
XposedHelpers.findAndHookMethod(aClass, "update", String.class, ContentValues.class, String.class, String[].class,new XC_MethodHook() {
@Override
protected void afterHookedMethod(MethodHookParam param) throws Throwable {
super.afterHookedMethod(param);
Log.e(TAG,"UpdateDBLOG");
Object arg = param.args[10];
}
});
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
} | efd6c3acfa9e3b1f62e07d6b6064f7e9056210e9 | [
"Markdown",
"Java"
] | 2 | Markdown | yb1994917/Awesome-XposedWechat | 7a325dcc0d80e7e228b71ac11ba19ccd5e62c6d1 | f1ea4da3bfb6288cf4603581321181032e50177a | |
refs/heads/master | <file_sep># Markdown Image caption.
Inspired by the probably un-maintained package [mdx_figcap](ttps://github.com/mkszk/mdx_figcap)
## Usage:
Make sure you have [markdown](https://pypi.org/project/Markdown/) installed.
Define an image with caption in your markdown.
```markdown

```
This will be converted to:
```html
<span class="img_container center" style="display: block;">
<img alt="test" src="http://example.com/image.png" style="display:block; margin-left: auto; margin-right: auto;" title="caption" />
<span class="img_caption" style="display: block; text-align: center;">caption</span>
</span>
```
Why no figure tag implementation ?
The figure tag is a block level element. The image element is an inline element. This difference breaks the attribute extension.
So `{: .center}` would not work if a figure was used.
## Installation
```bash
pip install markdown-image-caption
```
add the plugin to your markdown
```python
import markdown
parser = markdown.Markdown(extensions=["markdown_image_caption.plugin"])
```<file_sep>import markdown
import pytest
from markdown_image_caption.plugin import FigureCaptionExtension
import markdown.extensions.attr_list
input1 = ""
output1 = '<p><img alt="test" src="imgs/img.png" /></p>'
input2 = ''
output2 = '<p><span class="img_container" style="display: block;"><img alt="test" src="imgs/img.png" style="display:block; margin-left: auto; margin-right: auto;" title="figure caption" /><span class="img_caption" style="display: block; text-align: center;">figure caption</span></span></p>'
input3 = "no image here"
output3 = "<p>no image here</p>"
input4 = "{: .center}"
output4 = '<p><img alt="test" class="center" src="imgs/img.png" /></p>'
input5 = '{: .center}'
output5 = '<p><span class="img_container center" style="display: block;"><img alt="test" src="imgs/img.png" style="display:block; margin-left: auto; margin-right: auto;" title="figure caption" /><span class="img_caption" style="display: block; text-align: center;">figure caption</span></span></p>'
@pytest.fixture
def parser():
return markdown.Markdown(
extensions=[FigureCaptionExtension(), "markdown.extensions.attr_list"]
)
def test_image(parser):
txt = parser.convert(input1)
print(txt)
assert txt == output1
def test_figure(parser):
txt = parser.convert(input2)
print(txt)
assert txt == output2
def test_no_image(parser):
txt = parser.convert(input3)
assert txt == output3
def test_image_with_attr_list(parser):
txt = parser.convert(input4)
print(txt)
assert txt == output4
def test_figure_with_attr_list(parser):
txt = parser.convert(input5)
print(txt)
assert txt == output5
<file_sep>"""
Image Caption Extension for Python-Markdown
==================================
Converts \
to the image with a caption.
Its syntax is same as embedding images.
License: [BSD](http://www.opensource.org/licenses/bsd-license.php)
"""
from markdown.extensions import Extension
from markdown.inlinepatterns import IMAGE_LINK_RE
from markdown.util import etree
from markdown.inlinepatterns import ImageInlineProcessor
class FigureCaptionExtension(Extension):
def extendMarkdown(self, md):
# append to inline patterns
md.inlinePatterns["image_link"] = FigureCaptionPattern(
IMAGE_LINK_RE, md
)
class FigureCaptionPattern(ImageInlineProcessor):
def handleMatch(self, m, data):
image, start, index = super().handleMatch(m, data)
if image is None:
return image, start, index
title = image.get("title")
if title:
image.set(
"style",
"display:block; margin-left: auto; margin-right: auto;",
)
container = etree.Element(
"span",
attrib={"style": "display: block;", "class": "img_container"},
)
container.append(image)
etree.SubElement(
container,
"span",
attrib={
"class": "img_caption",
"style": "display: block; text-align: center;",
},
).text = title
return container, start, index
else:
return image, start, index
def makeExtension(*args, **kwargs):
return FigureCaptionExtension(*args, **kwargs)
| cd1b448d5d6ff64a14904847989140b6c3ff6f19 | [
"Markdown",
"Python"
] | 3 | Markdown | sander76/markdown-figure-caption | 0ffad4b30dca21ce530a24e7cc8ecf0f123772d1 | 35f29350a38b618e5f6813cb7f3b907b9919c0dc | |
refs/heads/master | <file_sep>from keras.datasets import mnist
from keras import backend as K
from keras.callbacks import TensorBoard
from keras.layers import Input, Dense, Convolution2D, MaxPooling2D, UpSampling2D
from keras.models import Model
import numpy as np
import matplotlib.pyplot as plt
def load_mnist():
(x_train, _), (x_test, _) = mnist.load_data()
x_train = x_train.astype('float32') / 255.
x_test = x_test.astype('float32') / 255.
#
# x_val = x_test[]
x_train = np.reshape(x_train, (len(x_train), 28, 28, 1))
x_test = np.reshape(x_test, (len(x_test), 28, 28, 1))
return x_train, x_test
def introduce_noise(x_train, x_test, noise_factor=0.5):
x_train_noisy = x_train + noise_factor * np.random.normal(
loc=0.0, scale=1.0, size=x_train.shape
)
x_test_noisy = x_test + noise_factor * np.random.normal(
loc=0.0, scale=1.0, size=x_test.shape
)
x_train_noisy = np.clip(x_train_noisy, 0., 1.)
x_test_noisy = np.clip(x_test_noisy, 0., 1.)
return x_train_noisy, x_test_noisy
def main():
# load the mnist data set and introduce some noise.
x_train, x_test = load_mnist()
x_train_noisy, x_test_noisy = introduce_noise(x_train, x_test)
# autoencoder part
input_img = Input(shape=(28, 28, 1))
x = Convolution2D(32, 3, 3, activation='relu', border_mode='same')(input_img)
x = MaxPooling2D((2, 2), border_mode='same')(x)
x = Convolution2D(32, 3, 3, activation='relu', border_mode='same')(x)
encoded = MaxPooling2D((2, 2), border_mode='same')(x)
x = Convolution2D(32, 3, 3, activation='relu', border_mode='same')(encoded)
x = UpSampling2D((2, 2))(x)
x = Convolution2D(32, 3, 3, activation='relu', border_mode='same')(x)
x = UpSampling2D((2, 2))(x)
decoded = Convolution2D(1, 3, 3, activation='sigmoid', border_mode='same')(x)
autoencoder = Model(input_img, decoded)
autoencoder.compile(optimizer='adadelta', loss='binary_crossentropy')
autoencoder.fit(x_train_noisy, x_train,
nb_epoch=1,
batch_size=128,
shuffle=True,
validation_split=1/12.,
callbacks=[TensorBoard(log_dir='/tmp/tb', histogram_freq=0,
write_graph=False)])
# decode (i.e. denoise) the images
decoded_imgs = autoencoder.predict(x_test_noisy)
n = 11
plt.figure(figsize=(20, 4))
for i in range(1, n):
# display original
ax = plt.subplot(2, n, i)
plt.imshow(x_test_noisy[100 + i].reshape(28, 28))
plt.gray()
ax.get_xaxis().set_visible(False)
ax.get_yaxis().set_visible(False)
# display reconstruction
ax = plt.subplot(2, n, i + n)
plt.imshow(decoded_imgs[100 + i].reshape(28, 28))
plt.gray()
ax.get_xaxis().set_visible(False)
ax.get_yaxis().set_visible(False)
plt.show()
if __name__ == '__main__':
main()
<file_sep>THEANO_FLAGS=device=gpu,floatX=float32 python3 vae_multi_view_faces.py
<file_sep>import numpy as np
from sklearn.neural_network import BernoulliRBM
from sklearn.datasets import load_digits
import matplotlib.pyplot as plt
def load_data():
"""
load training data and normalize
"""
digits = load_digits()
data = np.asarray(digits.data, dtype='float32')
training_data = data / np.max(data)
return training_data
def build_model(training_data):
"""
build and train the rbm.
"""
rbm = BernoulliRBM(random_state=0, verbose=True, n_components=100,
n_iter=50)
rbm.fit(training_data)
return rbm
def main():
rbm = build_model(load_data())
print(rbm.components_[0].shape)
# visualize
plt.figure(figsize=(5, 4.5))
for i, comp in enumerate(rbm.components_):
plt.subplot(10, 10, i + 1)
plt.imshow(comp.reshape((8, 8)), cmap=plt.cm.gray_r,
interpolation='nearest')
plt.xticks(())
plt.yticks(())
plt.suptitle('100 components extracted by RBM', fontsize=16)
plt.subplots_adjust(0.08, 0.02, 0.92, 0.85, 0.08, 0.23)
plt.show()
if __name__ == '__main__':
main()
<file_sep># unsupervised_deep_learning
Examples and implementations of unsupervised learning techniques used in the context of deep learning.
<file_sep>import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import norm
from keras.layers import Input, Dense, Lambda
from keras.models import Model
from keras import backend as K
from keras import objectives
from sklearn.cross_validation import train_test_split
from multi_view_faces import load_data
batch_size = 10
original_dim = 64 * 64
latent_dim = 2
intermediate_dim = 1024
nb_epoch = 8
epsilon_std = 1.0
x = Input(batch_shape=(batch_size, original_dim))
h = Dense(intermediate_dim, activation='relu')(x)
z_mean = Dense(latent_dim)(h)
z_log_var = Dense(latent_dim)(h)
def sampling(args):
z_mean, z_log_var = args
epsilon = K.random_normal(shape=(batch_size, latent_dim), mean=0.,
std=epsilon_std)
return z_mean + K.exp(z_log_var / 2) * epsilon
z = Lambda(sampling, output_shape=(latent_dim,))([z_mean, z_log_var])
decoder_h = Dense(intermediate_dim, activation='relu')
decoder_mean = Dense(original_dim, activation='sigmoid')
h_decoded = decoder_h(z)
x_decoded_mean = decoder_mean(h_decoded)
def vae_loss(x, x_decoded_mean):
xent_loss = original_dim * objectives.binary_crossentropy(x, x_decoded_mean)
kl_loss = - 0.5 * K.sum(1 + z_log_var - K.square(z_mean) - K.exp(z_log_var), axis=-1)
return xent_loss + kl_loss
vae = Model(x, x_decoded_mean)
vae.compile(optimizer='rmsprop', loss=vae_loss)
faces = load_data()
print(type(faces))
x_train, x_test = train_test_split(faces, test_size=0.1)
print(x_train)
x_train = x_train.astype('float32') / 255.
x_test = x_test.astype('float32') / 255.
x_train = x_train[:4100]
x_test = x_test[:450]
x_train = x_train.reshape((len(x_train), np.prod(x_train.shape[1:])))
x_test = x_test.reshape((len(x_test), np.prod(x_test.shape[1:])))
vae.fit(x_train, x_train,
shuffle=True,
nb_epoch=nb_epoch,
batch_size=batch_size,
validation_data=(x_test, x_test))
# build a model to project inputs on the latent space
encoder = Model(x, z_mean)
# sample from the learned distribution
decoder_input = Input(shape=(latent_dim,))
_h_decoded = decoder_h(decoder_input)
_x_decoded_mean = decoder_mean(_h_decoded)
generator = Model(decoder_input, _x_decoded_mean)
n = 15
face_size = 64
figure = np.zeros((face_size * n, face_size * n))
grid_x = norm.ppf(np.linspace(0.01, 0.99, n))
grid_y = norm.ppf(np.linspace(0.01, 0.99, n))
for i, yi in enumerate(grid_x):
for j, xi in enumerate(grid_y):
z_sample = np.array([[xi, yi]])
x_decoded = generator.predict(z_sample)
face = x_decoded[0].reshape(face_size, face_size)
figure[i * face_size: (i + 1) * face_size,
j * face_size: (j + 1) * face_size] = face
plt.figure(figsize=(10, 10))
plt.imshow(figure, cmap='Greys_r')
plt.show()
| d42479c47df96df54a6e44f508418c6214bd8e0c | [
"Markdown",
"Python",
"Shell"
] | 5 | Python | abiraja2004/unsupervised_deep_learning | 020214fb7bf8917b7b0b771d7aa54cf2c13bc07c | 91395235b73ed711f1d439c122ff04f67a589fd2 | |
refs/heads/master | <file_sep><?php
namespace Dwizzel\Routing;
error_reporting(E_ERROR);
Header('Content-type: text/html');
//------------------------------------------------------------------
class Debug{
public static function show($str){
echo '<pre>'.$str.'</pre>';
}
}
//------------------------------------------------------------------
class Routes{
/*
// php 7
protected static $routes = [
'home' => '/'
];
*/
protected static $routes = array(
'home' => '/'
);
private function get($key){
if(isset(static::$routes[$key])){
return static::$routes[$key];
}
return false;
}
private function set($key, $value){
static::$routes[$key] = $value;
}
public static function __callStatic($method, $args){
switch($method){
case 'get':
return (new Routes)->get($args[0]);
case 'set':
return (new Routes)->set($args[0], $args[1]);
default:
break;
}
}
}
//------------------------------------------------------------------
class Controller{
public function __construct(){
}
public function index(Request $request){
Debug::show(__METHOD__);
var_dump($request);
}
public function all(Request $request){
Debug::show(__METHOD__);
var_dump($request);
}
}
//------------------------------------------------------------------
class MiddleWare{
public function __construct(){
}
public function auth(Request $request){
Debug::show(__METHOD__);
var_dump($request);
$request->{'auth'} = true;
return $request;
}
public function process(Request $request){
Debug::show(__METHOD__);
var_dump($request);
$request->{'process'} = true;
return $request;
}
public function check(Request $request){
Debug::show(__METHOD__);
var_dump($request);
$request->{'check'} = true;
return $request;
}
public function verify(Request $request){
Debug::show(__METHOD__);
var_dump($request);
if($request->get('name') != 'dwizzel'){
Router::redirect(Routes::get('home'));
}
$request->{'verify'} = true;
return $request;
}
public function test(){
Debug::show(__METHOD__);
}
}
//------------------------------------------------------------------
class OuterWare{
public function in(Request $request){
Debug::show(__METHOD__);
var_dump($request);
$request->{'in'} = true;
}
public function middle(Request $request){
Debug::show(__METHOD__);
var_dump($request);
$request->{'middle'} = true;
}
public function out(Request $request){
Debug::show(__METHOD__);
var_dump($request);
$request->{'out'} = true;
}
}
//------------------------------------------------------------------
Class Request{
private $_data = array();
private $_method;
public function __construct(){
}
public function __set($key, $value){
$this->{$key} = $value;
}
public function __get($key){
if(isset($this->{$key})){
return $this->{$key};
}
return false;
}
public function set($key){
$this->_data = $value;
}
public function get($key){
if(isset($this->_data[$key])){
return $this->_data[$key];
}
return false;
}
public function data($method = 'get'){
$this->_method = $method;
foreach($_GET as $k=>$v){
$this->_data[$k] = $v;
}
switch($method){
case 'put':
parse_str(file_get_contents('php://input'), $_PUT);
foreach($_PUT as $k=>$v){
$this->_data[$k] = $v;
}
break;
case 'post':
foreach($_POST as $k=>$v){
$this->_data[$k] = $v;
}
break;
default:
break;
}
}
}
//------------------------------------------------------------------
Class Router{
protected static $inst;
protected static $request;
/*
// php 7
protected static $middleware = [
'out' => MiddleWare::class
];
*/
// php 5.4
protected static $outerware = array(
'in' => 'OuterWare',
'middle' => 'OuterWare',
'out' => 'OuterWare'
);
public function __construct(){
Debug::show(__METHOD__);
}
public static function redirect($url){
Header('Location: '.$url);
exit();
}
protected static function self($request = null){
self::$request = $request;
if (self::$inst === null){
self::$inst = new Router;
}
return self::$inst;
}
private function cleanUrl($value){
return ($value != '');
}
private function match($url, $path){
$paths = array_filter(explode('/', $path), 'self::cleanUrl');
$regs = array_filter(explode('/', $url), array(self, cleanUrl));
if(count($paths) != count($regs)){
return false;
}
$request = new Request();
var_dump(array($paths, $regs));
foreach($paths as $k=>$v){
if(!isset($regs[$k])){
return false;
}
$split = false;
//Debug::show('VALUE: "'.$v.'" :: "'.$regs[$k].'"');
if(preg_match('/^(.*)\{([a-zA-Z]+)\}(.*)$/', $regs[$k], $split)){
//print_r($split);
//Debug::show('REGEX: "/^'.$split[1].'([0-9a-zA-Z]+)'.$split[3].'$/"');
if(preg_match('/^'.$split[1].'([0-9a-zA-Z]+)'.$split[3].'$/', $v, $match)){
$request->{$split[2]} = $match[1];
}else{
return false;
}
}else if($regs[$k] != $v){
return false;
}
}
return $request;
}
private function callClassMethod($args){
list($class, $method) = explode('@', $args);
$class = __NAMESPACE__.'\\'.$class;
if(method_exists($class, $method)){
//$class::$method($req); //static :: deprecated
(new $class)->{$method}(self::$request);
}
}
private function callMiddleWare($arr){
foreach($arr as $k=>$v){
list($class, $method) = explode('@', $v);
$class = __NAMESPACE__.'\\'.$class;
if(method_exists($class, $method)){
self::$request = (new $class)->{$method}(self::$request);
if(self::$request === false){
return false;
}
}
}
return self::$request;
}
public static function __callStatic($method, $args){
Debug::show(__METHOD__);
if(self::$request != null){
return;
}
//var_dump($args);
if(strtoupper($method) != $_SERVER['REQUEST_METHOD']){
return;
}
//(new Router)->self();
$url = parse_url($_SERVER['REQUEST_URI']);
$path = $url['path'];
$match = $args[0];
$func = $args[1];
self::self(self::match($match, $path));
if(self::$request){
self::$request->data($method);
if(isset($args[2]) && is_array($args[2])){
self::$request = self::callMiddleWare($args[2]);
if(self::$request === false){
//return self::$inst;
//return new static;
return;
}
}
(is_string($func))? self::callClassMethod($func) : $func(self::$request);
}
//return self::$inst;
//return new static;
return;
}
public function chain($name){
if(is_array($name)){
foreach($name as $v){
$class = __NAMESPACE__.'\\'.$outerware[$v];
(new self::$class)->{$v}(self::$request);
}
}else{
$class = __NAMESPACE__.'\\'.$outerware[$name];
(new self::$class)->{$name}(self::$request);
}
return self::$inst;
}
public function __call($method, Array $args){
Debug::show('dynamic method "'.$method.'" dont exist');
}
}
//------------------------------------------------------------------
Routes::set('clients', '/clients/');
Routes::set('clients-0', '/clients/');
Routes::set('test-0', '/test-0/');
Routes::set('test-1', '/test-1/');
Routes::set('test-2', '/test-2/');
Routes::set('clients-id', '/clients/{id}/');
Routes::set('clients-account-id', '/clients/{id}/accounts/{accountId}/');
//(new Router)->test();
Router::get('/', 'Controller@index');
Router::get('/clients/', 'Controller@all',
['MiddleWare@auth','MiddleWare@check', 'MiddleWare@process']);
Router::get('/clients/{id}/', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
}, ['MiddleWare@check', 'MiddleWare@process']);
Router::get('/clients/{id}/accounts/{accountId}/', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
}, ['MiddleWare@verify']);
Router::get('/clients/{id}/[a-z]{3}-{accountId}-test/', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
});
Router::get('/clients/{id}/{accountId}/', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
});
// -- testing -------------------------------
Router::get('/test-0/', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
});
Router::get('/test-1/', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
}, ['MiddleWare@auth']);
Router::get('/test-2/', 'Controller@index', ['MiddleWare@auth']);
Router::get('/clients-0/', function (Request $request){
Debug::show(__METHOD__);
var_dump($request);
}, ['MiddleWare@auth']);
// - get, update, delete -----------------------------
Router::put('/clients/{id}', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
});
Router::delete('/clients/{id}', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
});
Router::post('/clients/', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
});
<file_sep><?php
namespace Dwizzel\Routing;
error_reporting(E_ALL);
//Header('Content-type: text/html');
//------------------------------------------------------------------
class Debug{
public static function show($str){
echo '<pre>'.$str.'</pre>';
}
}
//------------------------------------------------------------------
class Routes{
/*
// php 7
protected static $routes = [
'home' => '/'
];
*/
protected static $routes = array(
'home' => '/'
);
private function get($key){
if(isset(static::$routes[$key])){
return static::$routes[$key];
}
return false;
}
private function set($key, $value){
static::$routes[$key] = $value;
}
public static function __callStatic($method, $args){
switch($method){
case 'get':
return (new Routes)->get($args[0]);
case 'set':
return (new Routes)->set($args[0], $args[1]);
default:
break;
}
}
}
//------------------------------------------------------------------
class Controller{
public function __construct(){
}
public function index(Request $request){
Debug::show(__METHOD__);
var_dump($request);
}
public function all(Request $request){
Debug::show(__METHOD__);
var_dump($request);
}
}
//------------------------------------------------------------------
class MiddleWare{
public function __construct(){
}
public function auth(Request $request){
Debug::show(__METHOD__);
var_dump($request);
$request->{'auth'} = true;
return $request;
}
public function process(Request $request){
Debug::show(__METHOD__);
var_dump($request);
$request->{'process'} = true;
return $request;
}
public function check(Request $request){
Debug::show(__METHOD__);
var_dump($request);
$request->{'check'} = true;
return $request;
}
public function verify(Request $request){
Debug::show(__METHOD__);
var_dump($request);
if($request->get('name') != 'dwizzel'){
Router::redirect(Routes::get('home'));
}
$request->{'verify'} = true;
return $request;
}
public function test(){
Debug::show(__METHOD__);
}
}
//------------------------------------------------------------------
class OuterWare{
public function in(Response $response){
Debug::show(__METHOD__);
$response->set('in', true);
return $response;
}
public function middle(Response $response){
Debug::show(__METHOD__);
$response->set('middle', true);
return $response;
}
public function out(Response $response){
Debug::show(__METHOD__);
$response->set('out', true);
return $response;
}
}
//------------------------------------------------------------------
class Json{
static public function encode($data){
if (function_exists('json_encode')) {
$data = json_encode($data, JSON_UNESCAPED_UNICODE);
if(json_last_error() != JSON_ERROR_NONE){
return json_last_error();
}
return $data;
}
return false;
}
static public function decode($json, $assoc = false){
if (function_exists('json_decode')) {
$data = json_decode($json, true);
if(json_last_error() != JSON_ERROR_NONE){
return json_last_error();
}
return $data;
}
return false;
}
}
//------------------------------------------------------------------
class Response{
private $_data = array();
private $_headers = array();
private $_errors = array();
public function __construct(){
}
public function set($key, $value){
$this->_data[$key] = $value;
}
public function get($key){
if(isset($this->_data[$key])){
return $this->_data[$key];
}
return false;
}
public function sets($values){
if(is_array($values)){
$this->_data = $this->recursiveSet($this->_data, $values);
return true;
}
return false;
}
private function recursiveSet($arr, $values){
if(is_array($values)){
foreach($values as $k=>$v){
if(is_array($v)){
$arr[$k] = array();
$this->recursiveSet($arr[$k], $v);
}else{
$arr[$k] = $v;
}
}
}
return $arr;
}
public function clear($bErrors = false) {
$this->_data = array();
if($bErrors){
$this->_errors = array();
}
}
public function addError($errmsg, $errno = 0) {
array_push($this->_errors, array(
'code' => $errno,
'message' => $errmsg
));
}
public function sendHeader($html = false) {
if(!headers_sent()) {
$this->addHeader('Cache-Control:no-cache, private');
if($html){
$this->addHeader('Content-Type: text/html; charset=utf-8');
}else{
$this->addHeader('Content-Type: application/json; charset=utf-8');
}
foreach ($this->_headers as $header) {
header($header, true);
}
}
}
public function addHeader($header) {
$this->_headers[] = $header;
}
public function output(){
if(count($this->_errors)){
$this->addHeader('HTTP/1.0 400 Bad Request');
$this->_data = $this->_errors;
}
$encoded = Json::encode($this->_data);
if($encoded === false || is_numeric($encoded)){
$this->clear(true);
$this->addError('json error: '.$encoded);
$this->output();
return false;
}
return $encoded;
}
}
//------------------------------------------------------------------
class Request{
private $_data = array();
private $_method;
public function __construct(){
}
public function __set($key, $value){
$this->{$key} = $value;
}
public function __get($key){
if(isset($this->{$key})){
return $this->{$key};
}
return false;
}
public function set($key, $value){
$this->_data[$key] = $value;
}
public function get($key){
if(isset($this->_data[$key])){
return $this->_data[$key];
}
return false;
}
public function getData(){
return $this->_data;
}
public function data($method = 'get'){
$this->_method = $method;
foreach($_GET as $k=>$v){
$this->_data[$k] = $v;
}
switch($method){
case 'put':
parse_str(file_get_contents('php://input'), $_PUT);
foreach($_PUT as $k=>$v){
$this->_data[$k] = $v;
}
break;
case 'post':
foreach($_POST as $k=>$v){
$this->_data[$k] = $v;
}
break;
default:
break;
}
}
}
//------------------------------------------------------------------
class Router{
protected static $inst = null;
protected static $request = null;
protected static $response = null;
public function __construct(){
Debug::show(__METHOD__);
}
public static function redirect($url){
//Debug::show(__METHOD__);
Header('Location: '.$url);
exit();
}
protected static function self($request = null){
//Debug::show(__METHOD__);
self::$request = $request;
if (self::$inst === null){
self::$inst = new Router;
}
return self::$inst;
}
private function cleanUrl($value){
//Debug::show(__METHOD__);
return ($value != '');
}
private function match($url, $path){
//Debug::show(__METHOD__);
$paths = array_filter(explode('/', $path), 'self::cleanUrl');
$regs = array_filter(explode('/', $url), 'self::cleanUrl');
if(count($paths) != count($regs)){
return;
}
$request = new Request;
var_dump(array($paths, $regs));
foreach($paths as $k=>$v){
if(!isset($regs[$k])){
return;
}
$split = false;
//Debug::show('VALUE: "'.$v.'" :: "'.$regs[$k].'"');
if(preg_match('/^(.*)\{([a-zA-Z]+)\}(.*)$/', $regs[$k], $split)){
//Debug::show('REGEX: "/^'.$split[1].'([0-9a-zA-Z]+)'.$split[3].'$/"');
if(preg_match('/^'.$split[1].'([0-9a-zA-Z]+)'.$split[3].'$/', $v, $match)){
$request->{$split[2]} = $match[1];
}else{
return;
}
}else if($regs[$k] != $v){
return;
}
}
return $request;
}
private function callClassMethod($args){
Debug::show(__METHOD__);
list($class, $method) = explode('@', $args);
$class = __NAMESPACE__.'\\'.$class;
if(method_exists($class, $method)){
//$class::$method($req); //static :: deprecated
(new $class)->{$method}(self::$request);
}
}
private function callMiddleWare($arr){
Debug::show(__METHOD__);
foreach($arr as $k=>$v){
list($class, $method) = explode('@', $v);
$class = __NAMESPACE__.'\\'.$class;
if(method_exists($class, $method)){
self::$request = (new $class)->{$method}(self::$request);
if(self::$request === false){
return false;
}
}
}
return true;
}
public static function __callStatic($method, $args){
//Debug::show(__METHOD__);
if(strtoupper($method) != $_SERVER['REQUEST_METHOD']){
return self::$inst;
}
if(!is_null(self::$request)){
return self::$inst;
}
//(new Router)->self();
$url = parse_url($_SERVER['REQUEST_URI']);
$path = $url['path'];
$match = $args[0];
$func = $args[1];
self::self();
self::$request = self::$inst->match($match, $path);
if(self::$request){
self::$response = new Response;
self::$request->data($method);
if(isset($args[2]) && is_array($args[2])){
if(!self::$inst->callMiddleWare($args[2])){
return self::$inst;
}
}
(is_string($func))? self::$inst->callClassMethod($func) : $func(self::$request);
}
return self::$inst;
}
private function chain($name){
Debug::show(__METHOD__);
if(is_array($name)){
foreach($name as $v){
list($class, $method) = explode('@', $v);
$class = __NAMESPACE__.'\\'.$class;
self::$response = (new $class)->{$method}(self::$response);
}
}else{
list($class, $method) = explode('@', $name);
$class = __NAMESPACE__.'\\'.$class;
self::$response = (new $class)->{$method}(self::$response);
}
return self::$response;
}
public function end($name = null){
//Debug::show(__METHOD__);
if(is_null(self::$response)){
return self::$inst;
}
if($name !== null){
self::$inst->chain($name);
}
self::$response->set('done', 'OK');
self::$response->sets(self::$request->getData());
self::$response->sendHeader(true);
echo self::$response->output();
exit();
}
public function __call($method, Array $args){
Debug::show(__METHOD__);
Debug::show('dynamic method "'.$method.'" dont exist');
}
}
//------------------------------------------------------------------
Routes::set('clients', '/clients/');
Routes::set('clients-id', '/clients/{id}/');
Routes::set('clients-account-id', '/clients/{id}/accounts/{accountId}/');
//(new Router)->test();
Router::get('/', 'Controller@index')->end();
Router::get('/clients/', 'Controller@all', ['MiddleWare@check', 'MiddleWare@process'])
->end(['OuterWare@in', 'OuterWare@middle', 'OuterWare@out']);
Router::get('/clients/{id}/', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
}, ['MiddleWare@auth', 'MiddleWare@check', 'MiddleWare@process'])->end('OuterWare@out');
Router::get('/clients/{id}/accounts/{accountId}/', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
}, ['MiddleWare@verify'])->end();
Router::get('/clients/{id}/[a-z]{3}-{accountId}-test/', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
})->end();
Router::get('/clients/{id}/{accountId}/', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
})->end();
// - get, update, delete -----------------------------
Router::put('/clients/{id}', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
});
Router::delete('/clients/{id}', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
});
Router::post('/clients/', function(Request $request){
Debug::show(__METHOD__);
var_dump($request);
});
| 3423e2b58bc911b57d315c3d12083d545a3336b2 | [
"PHP"
] | 2 | PHP | dwizzel-dev/router-test | a6e156c26e99c3dd9f981b6eb43e68f6df3b15b8 | 2019e3bca23371ab1e1bfc55d343d6f3ebd40a63 | |
refs/heads/master | <repo_name>jackdreilly/commroute<file_sep>/src/ctm.py
from cr_utils import Dumpable
from cr_network import Link
from demand import FlowNetwork
class FundamentalDiagram(Dumpable):
"""docstring for FundamentalDiagram"""
def __init__(self, v, w, rho_max, q_max):
super(FundamentalDiagram, self).__init__()
self.v = float(v)
self.w = float(w)
self.rho_max = float(rho_max)
self.q_max = float(q_max)
def jsonify(self):
"""docstring for jsonify"""
return {
'v': self.v,
'w': self.w,
'rho_max': self.rho_max,
'q_max': self.q_max
}
def rho_cong(self, flow):
return self.rho_max - float(flow)/self.w
def flow_cong(self, rho):
return self.w * (self.rho_max - rho)
def rho_ff(self, flow):
return float(flow) / self.v
def flow_ff(self, rho):
return self.v * rho
def flow(self, rho):
return min(self.q_max, self.flow_cong(rho), self.flow_ff(rho))
@classmethod
def load_with_json_data(cls, data):
return cls(
v=data['v'],
w=data['w'],
q_max=data['q_max'],
rho_max=data['rho_max']
)
class CTMLink(Link):
"""docstring for CTMLink"""
def __init__(self, l, fd, flow=0.0, *args, **kwargs):
super(CTMLink, self).__init__(*args, **kwargs)
self.l = l
self.fd = fd
self.flow = flow
def jsonify(self):
json = super(CTMLink, self).jsonify()
json.update({
'l': self.l,
'fd': self.fd.jsonify(),
'flow': self.flow
})
return json
def d3_value(self):
return self.l
class DensityCTMLink(CTMLink):
"""docstring for DensityCTMLink"""
def __init__(self, rho=0.0, *args, **kwargs):
super(DensityCTMLink, self).__init__(*args, **kwargs)
self.rho = rho
def jsonify(self):
"""docstring for jsonify"""
json = super(DensityCTMLink, self).jsonify()
json.update({
'rho': self.rho
})
return json
@classmethod
def additional_kwargs(cls, data):
return dict(
l=data['l'],
rho=data['rho'],
flow=data['flow'],
fd=FundamentalDiagram.load_with_json_data(data['fd'])
)
class DensityCTMNetwork(FlowNetwork):
link_class = DensityCTMLink
def __init__(self):
super(DensityCTMNetwork, self).__init__()
def tt_free_flow(self, route):
return sum(link.l / link.fd.v for link in route.get_links)
if __name__ == '__main__':
print 'hei'
<file_sep>/src/static_op.py
from cr_optimize import SimpleOptimizeMixIn
from ctm import *
from cvxpy import variable, eq, geq
from demand import ODDemand, RouteDemand
class LagrangianStaticProblem(FlowNetwork, SimpleOptimizeMixIn):
"""docstring for StaticProblem"""
def cvxify(self):
"""docstring for cvxify"""
self.cache_props()
for link in self.get_links():
link.v_flow = variable(name='flow: {0}'.format(link.name))
for (source, sink), routes in self.od_routes.iteritems():
for i, route in enumerate(routes):
route.v_flow = variable(name='rf: o: {0}, d: {1} [{2}]'.format(source.name, sink.name, i))
class LagrangianConstrained(LagrangianStaticProblem):
def con_junc(self):
return [eq(sum(link.v_flow for link in junction.in_links),
sum(link.v_flow for link in junction.out_links))
for junction in self.junctions]
def con_od_flows(self):
od_demands = filter(lambda dem: isinstance(dem, ODDemand), self.demands)
route_demands = filter(lambda dem: isinstance(dem, RouteDemand), self.demands)
def route_flows(link):
flow = 0.0
link_routes = link.routes()
for route in link_routes:
flow += route.v_flow
for dem in route_demands:
if dem.route in link_routes:
flow += dem.flow
return flow
def od_flows(source, sink):
flow = 0
for route in self.od_routes[source, sink]:
flow += route.v_flow
return flow
return [
eq(route_flows(link), link.v_flow)
for link in self.get_links()
] + [
eq(od_flows(dem.source, dem.sink), dem.flow)
for dem in od_demands
] + [
geq(route.v_flow, 0.0) for route in self.all_routes()
]
def constraints(self):
return super(LagrangianConstrained, self).constraints() + self.con_od_flows()<file_sep>/src/static_ctm.py
from cvxpy import geq, leq, quad_over_lin, variable, hstack, minimize, eq
from cvxpy import max as cvx_max
from cr_optimize import SimpleOptimizeMixIn
from cr_utils import flatten
from ctm import DensityCTMNetwork
from static_op import LagrangianConstrained
from numpy import inf
__author__ = 'jdr'
class CTMStaticProblem(DensityCTMNetwork, LagrangianConstrained):
def cvxify(self):
super(CTMStaticProblem, self).cvxify()
self.cache_props()
for link in self.get_links():
link.v_dens = variable(name='dens: {0}'.format(link.name))
# this next line isn't useful right now
# link.d3_value = lambda: link.v_dens.value
class CTMConstrained(CTMStaticProblem):
def con_ctm(self):
return list(flatten([geq(link.v_flow, 0),
leq(link.v_flow, link.fd.q_max),
leq(link.v_flow, link.fd.v * link.v_dens),
leq(link.v_flow, link.fd.w * (link.fd.rho_max - link.v_dens)),
geq(link.v_dens, 0),
leq(link.v_dens, link.fd.rho_max),
] for link in self.get_links()))
def constraints(self):
return super(CTMConstrained, self).constraints() + self.con_ctm()
def check_feasible_constraints(self):
return list(flatten(
[geq(link.v_flow, link.flow), geq(link.v_dens, link.rho),leq(link.v_flow, link.flow), leq(link.v_dens, link.rho)]
for link in self.get_links()
))
def check_feasible(self):
class FeasibleProgram(SimpleOptimizeMixIn):
outer = self
def constraints(self):
return self.outer.constraints() + self.outer.check_feasible_constraints()
def cvxify(self):
self.outer.cvxify()
op = FeasibleProgram()
prog = op.get_program()
prog.show()
return not (prog.solve(quiet=True) == inf)
class ComplianceConstrained(CTMConstrained):
def route_tt_heuristic(self, route):
def link_tt_heuristic(link):
ff = link.l / link.fd.v
q_max = (link.l / link.fd.q_max) * link.v_dens
rho_hat = link.fd.rho_max - link.v_dens
cong = link.l / link.fd.w * (quad_over_lin(link.fd.rho_max ** .5, rho_hat) - 1)
return cvx_max(hstack([ff, q_max, cong]))
return sum(map(link_tt_heuristic, route.links))
def route_tt_real(self, route):
def link_tt(link):
if link.v_dens.value < link.fd.rho_max * 10e-8:
return link.l / link.fd.v
return link.l * link.v_dens.value / link.v_flow.value
return sum([
link_tt(link)
for link in route.get_links
])
def con_route_tt(self):
return [
leq(self.route_tt_heuristic(route), 10000.0)
for route in self.all_routes()
]
def constraints(self):
return super(ComplianceConstrained, self).constraints() + self.con_route_tt()
class MinTTT(CTMStaticProblem):
def objective(self):
return minimize(sum(link.l * link.v_dens for link in self.get_links()))
class MinTTTComplianceProblem(MinTTT, ComplianceConstrained):
def __init__(self):
super(MinTTTComplianceProblem, self).__init__()
def objective(self):
return MinTTT.objective(self)
def constraints(self):
return ComplianceConstrained.constraints(self)
class MinTTTLagrangianCTMProblem(MinTTT, CTMConstrained):
def __init__(self):
super(MinTTTLagrangianCTMProblem, self).__init__()
def objective(self):
return MinTTT.objective(self)
def constraints(self):
return CTMStaticProblem.constraints(self)<file_sep>/src/cr_utils.py
__author__ = 'jdr'
from simplejson import dump, load
from itertools import chain
class Dumpable(object):
def dump(self, fn):
"""docstring for dump"""
with open(fn, 'w') as fn:
dump(self.jsonify(), fn, indent=2)
def jsonify(self):
raise NotImplementedError('need to jsonify!')
@classmethod
def load(cls, fn, **kwargs):
with open(fn, 'r') as fn:
data = load(fn)
return cls.load_with_json_data(data, **kwargs)
@classmethod
def load_with_json_data(cls, data, **kwargs):
raise NotImplementedError('need to load object!')
def flatten(listOfLists):
"Flatten one level of nesting"
return chain.from_iterable(listOfLists)
<file_sep>/src/experiments.py
from demand import RouteDemand, ODDemand
__author__ = 'jdr'
from static_ctm import *
from ctm import FundamentalDiagram
from ctm import DensityCTMLink
from cr_network import Junction
def exp_1_create():
"""
create the base network
"""
v = 1.0
w = .5
rho_max = 3
q_max = 1.0
l = 1.0
fd = FundamentalDiagram(
v=v,
w=w,
rho_max=rho_max,
q_max=q_max
)
net = DensityCTMNetwork()
source = DensityCTMLink(
net=net,
name='source',
l=l,
fd=fd,
rho=1.0
)
left = DensityCTMLink(
net=net,
name='left',
l=l,
fd=fd,
rho=1.0
)
right = DensityCTMLink(
net=net,
name='right',
l=2 * l,
fd=fd,
rho=1.0
)
sink = DensityCTMLink(
net=net,
name='sink',
l=l,
fd=fd,
rho=1.0
)
junctions = [
Junction([source], [left, right]),
Junction([left, right], [sink]),
]
for junction in junctions:
net.add_junction(junction)
net.dump("networks/exps/exp1/net.json")
def exp_1_demands():
net = DensityCTMNetwork.load('networks/exps/exp1/net.json')
left = net.route_by_names([
'source', 'left', 'sink'
])
route_demand = RouteDemand(left, .2)
od_demand = ODDemand(net.link_by_name('source'), net.link_by_name('sink'), .8)
net.demands.extend([route_demand, od_demand])
net.dump('networks/exps/exp1/net_w_demand.json')
def exp_1_opt():
'''
@return: nothing, demonstrate
show that you can add constraints on the fly, and that this increases the objective when you force large densities
'''
net = MinTTTComplianceProblem.load('networks/exps/exp1/net_w_demand.json')
prog = net.get_program()
prog.show()
prog.solve()
print 'obnjective', prog.objective.value
left = net.link_by_name('left')
prog.constraints.extend([
geq(left.v_dens, left.fd.v * left.fd.q_max * 1.2)
])
prog.show()
prog.solve()
print 'obnjective', prog.objective.value
for link in net.get_links():
link.flow = link.v_flow.value
link.rho = link.v_dens.value
net.d3ize()
print net.check_feasible()
def exp_1_nash():
net = MinTTTComplianceProblem.load('networks/exps/exp1/net_w_demand.json')
left = net.link_by_name('left')
right = net.link_by_name('right')
left.flow = .75
left.rho = left.fd.rho_cong(left.flow)
right.flow = 1 - left.flow
right.rho = right.fd.rho_ff(right.flow)
source = net.link_by_name('source')
sink = net.link_by_name('sink')
source.flow = 1.0
source.rho = source.fd.rho_ff(source.flow)
sink.flow = 1.0
sink.rho = source.fd.rho_ff(sink.flow)
net.dump('networks/exps/exp1/net_nash.json')
def exp_1_nash_feasible():
net = MinTTTComplianceProblem.load('networks/exps/exp1/net_nash.json')
print net.check_feasible()
def main():
# exp_1_create()
# exp_1_demands()
# exp_1_opt()
# exp_1_nash()
exp_1_nash_feasible()
if __name__ == '__main__':
main()
<file_sep>/src/cr_optimize.py
from cr_utils import Dumpable
from cr_network import CRNetwork
from demand import Demand
from cvxpy import program, minimize
__author__ = 'jdr'
class OptimizeMixIn(object):
def constraints(self):
raise NotImplementedError("implement me")
def objective(self):
raise NotImplementedError("implement me")
def cvxify(self):
pass
def get_program(self):
self.cvxify()
return program(self.objective(), self.constraints())
def solve_problem(self):
program = self.get_program()
program.solve()
return program
class Feasible:
def objective(self):
return minimize(0.0)
class Unconstrained:
def constraints(self):
return []
class SimpleOptimizeMixIn(Feasible, Unconstrained, OptimizeMixIn):
pass | fcc8a446c5f12e1fad291178476b7079762ad289 | [
"Python"
] | 6 | Python | jackdreilly/commroute | 1e0fca4d866d6e445bfb87e894c7c1ee007db47f | ed0b9a079c5ff33b53b0ffb8eb48e48f4ae8bfd1 | |
refs/heads/main | <repo_name>kss5982/rock-paper-scissors<file_sep>/rps-script.js
function computerPlay() {
let randomInt = Math.floor(Math.random() * 3)
if (randomInt == 0) {
return "rock"
} else if (randomInt == 1) {
return "paper"
} else {
return "scissors"
}
}
function playRound(playerSelection, computerSelection) {
let playerSelectionLower = playerSelection.toLowerCase()
if ((playerSelectionLower == "rock" && computerSelection == "scissors") || (playerSelectionLower == "paper" && computerSelection == "rock") || (playerSelectionLower == "scissors" && computerSelection == "paper")) {
console.log("You win this round! " + playerSelection + " beats " + computerSelection)
return 1
} else if ((computerSelection == "rock" && playerSelectionLower == "scissors") || (computerSelection == "paper" && playerSelectionLower == "rock") || (computerSelection == "scissors" && playerSelectionLower == "paper")) {
console.log("You lost this round! " + computerSelection + " beats " + playerSelection)
return -1
} else {
console.log("Draw!")
}
}
function game() {
let playerScore = 0
let computerScore = 0
let counter = 0
for (let i = 0; i < 5; i++) {
const playerSelection = prompt("Please make your choice: ")
const computerSelection = computerPlay();
counter = playRound(playerSelection, computerSelection)
if (counter == 1) {
playerScore++
} else if (counter == -1) {
computerScore++
}
}
if (playerScore > computerScore) {
console.log("You won!")
} else if (computerScore < playerScore) {
console.log("You lose!")
} else {
console.log("Tie game!")
}
}
game() | 159455194017a21f9895d3a9cd337e6c9bd4b656 | [
"JavaScript"
] | 1 | JavaScript | kss5982/rock-paper-scissors | d4e7be4acd9646fa276cb0c1ad88976c6417a09b | 074a5171399679aa78f9a848ddf3534639107785 | |
refs/heads/master | <file_sep>/* Sam (Sean) <NAME>
* Capitalize the first letter of every word in a string of words
* Java version "1.8.0_201"
* Java(TM) SE Runtime Environment (build 1.8.0_201-b09)
* Java HotSpot(TM) 64-Bit Server VM (build 25.201-b09, mixed mode)
* February 18th, 2019
* */
import java.util.Scanner;
public class capitalize {
public static void main(String[] args){
//variables needed
String input;
String[] words;
String output = "";
//scanner for user input
Scanner sc = new Scanner(System.in);
//prompt user and store result in words
System.out.println("Enter a string of words: ");
input = sc.nextLine();
//split the input and seperate at the spaces, could add other delimiters.
words = input.split("\\s+");
for(int i = 0; i < words.length; ++i){
//replace the first character with a capital, keep the rest intact
words[i] = words[i].substring(0,1).toUpperCase() + words[i].substring(1);
//
output += words[i] + " ";
}
//print output
System.out.println(output);
}
}
<file_sep>/*
<NAME>
Prime number generator
Current TODOs:
-write to file
*/
/*standard output*/
#include <stdio.h>
/*string to int conversion*/
#include <stdlib.h>
/*Timing*/
#include <time.h>
/*Check command line flags*/
#include <string.h>
/*Multithreading*/
#include <omp.h>
int bruteforceInt(long int checkNumber);
int fastbruteforceInt(long int checkNumber);
int main(int argc, char **argv){
/*Calculator option variables*/
long int STARTING_POINT_CALC = 1;
long int ENDING_POINT_CALC = 5000000;
long int number;
/*Thread variables*/
int MULTITHREAD = 0;
/*Clock Variables*/
int NUM_OF_THREADS = 4;
clock_t begin, end;
double time_spent;
/*Flag variables*/
int temp;
int flags[3];
/*check flags*/
for(temp = 0; temp < argc; ++temp){
/*fast bruteforce*/
if(strcmp(argv[temp], "-fast") == 0) flags[0] = 1;
/*enable clock*/
if(strcmp(argv[temp], "-time") == 0) flags[1] = 1;
/*write to file TODO*/
if(strcmp(argv[temp], "-write") == 0) flags[2] = 1;
/*enable threading*/
if(strcmp(argv[temp], "-thread") == 0) MULTITHREAD = 1;
/*change start point*/
if(strcmp(argv[temp], "-start") == 0){
STARTING_POINT_CALC = atoi(argv[temp+1]);
++temp;
}
/*change end point*/
if(strcmp(argv[temp], "-end") == 0){
ENDING_POINT_CALC = atoi(argv[temp+1]);
++temp;
}
}
/*start clock*/
if(flags[1]==1){
begin = clock();
}
/*start multithreading*/
#pragma omp parallel if(MULTITHREAD)
/*start calculating*/
if(flags[0]==1){
for(number = STARTING_POINT_CALC; number < ENDING_POINT_CALC; ++number){
fastbruteforceInt(number);
}
} else {
for(number = STARTING_POINT_CALC; number < ENDING_POINT_CALC; ++number){
bruteforceInt(number);
}
}
/*end clock and calculate time*/
if(flags[1]==1){
end = clock();
time_spent = (double)(end - begin) / (CLOCKS_PER_SEC * NUM_OF_THREADS);
printf("Time spent: %f seconds\n", time_spent);
}
return 0;
}
int bruteforceInt(long int checkNumber){
long int i;
int prime = 1;
/*checks every single possible devision statement to see if it gives a remainder. If it doesn't, checkNumber is prime*/
for(i = 1; i < checkNumber; ++i){
if(checkNumber % i == 0 && i != 1){
prime = 0;
}
}
if(prime == 1 && checkNumber != 1){
printf("%ld\n", checkNumber);
}
return 0;
}
int fastbruteforceInt(long int checkNumber){
long int i,j;
int prime = 1;
/*checks devision statement to see if it gives a remainder. If it doesn't, checkNumber is prime and end condition is set to answer + 1.*/
for(i = 1, j = checkNumber; i < j; ++i){
if(checkNumber % i == 0 && i != 1){
prime = 0;
break;
}
else {
/*Weird case, doesnt work with 2.*/
if(checkNumber != 2){
j = (checkNumber/i) + 1;
}
}
}
if(prime == 1 && checkNumber != 1){
printf("%ld\n", checkNumber);
}
return 0;
}
<file_sep>#Sam (<NAME>
#Capitalize the first letter of every word in a string of words
#Python 3.6.7
#February 18th, 2019
#prompt user for a string of words
text = input("Enter a string of words: ")
#Split Strings by spaces. Other cases can be added. Example included
#words.replace(',', ' ') #uncomment to add punctuation case for ','. other cases can be added
words = text.split()
newString = ""
#loop through each word that was split
for words in words:
#capitalize the word
words = words.capitalize()
#Add the string to the new string
newString += words + " "
#print the result
print(newString)
<file_sep>/* <NAME>
* A simple bubble sort I made
* While waiting on my friends to finish their other work
* February 15th, 2019
*/
#include <stdio.h>
int main(){
int i, j;
int temp = 0;
int numArray[15] = {8,10,42,1,96,54,22,88,51,62,42, 88, 100, 3, 29};
/*Print the initial array*/
printf("initial array: ");
for(i = 0; i < 15; ++i){
printf("%d ", numArray[i]);
}
printf("\n");
/*Bubble Sort*/
for(i = 0; i < 15; ++i){
for(j = i; j < 15; ++j){
/*Swap spots in array*/
if(numArray[j] < numArray[i]){
temp = numArray[i];
numArray[i] = numArray[j];
numArray[j] = temp;
}
}
}
/*Print sorted array*/
printf("sorted array: ");
for(i = 0; i < 15; ++i){
printf("%d ", numArray[i]);
}
printf("\n");
return 0;
}
<file_sep>all: fibonacci prime permute bubblesort
fibonacci:
gcc -Wall -ansi -pedantic fibonacci.c -o fibonacci
prime:
gcc -fopenmp -Wall -ansi -pedantic prime.c -o prime
permute:
gcc -Wall -ansi -pedantic permute.c -o permute
bubblesort:
gcc -Wall -ansi -pedantic bubblesort.c -o bubblesort
clean:
rm prime fibonacci permute bubblesort
<file_sep>/* <NAME>
* January 13th, 2019
* prints all possible permutations of the characters in a word
* Modified version of my school work to work for more words.
* */
#include <stdio.h>
#include <string.h>
/*function prototypes*/
void permute(char *word);
void recursiveCharacters(char *word, int index);
void swap(char *a, char *b);
/*function definitions*/
int main(int argc, char** argv){
if(argc == 1){
printf("Not enough arguments. format is \"./permute <word_to_be_permutated>\".\n");
} else if(argc >= 3) {
printf("Too many arguments. format is \"./permute <word_to_be_permutated>\".\n");
} else {
permute(argv[1]);
}
return 0;
}
/* gets called from main, starts the recursive function
* could also be called from an external function call in the future*/
void permute(char *word){
recursiveCharacters(word, 0);
return;
}
/*get every permutation of the word using recursion*/
void recursiveCharacters(char *word, int index){
int i;
int end = strlen(word) - 1;
/*check if each character has been swapped*/
if(index != end){
for(i = index; i <= end; ++i){
/*swap characters for a new combination*/
swap(word + index, word + i);
/*call the recursive function, increment the index*/
recursiveCharacters(word, index + 1);
/*swap the characters back so it doesn't mess up later combinations*/
swap(word + index, word + i);
}
} else {
/*print the word once 6 letters have swapped*/
/*printWordTest(word);*/
printf("%s\n", word);
}
return;
}
/*Fairly standard swap function for strings*/
void swap(char *a, char *b){
char temp;
temp = *a;
*a = *b;
*b = temp;
return;
}
<file_sep>#<NAME>
#A REALLY simple quadratic calculator
#to assist in learning python
import math
#using python 2
print "Quadratic Root Calculator"
print "Enter the coefficients (format AX^2 + BX + C)"
#get user input
A = raw_input('AX^2:')
A = int(A)
B = raw_input('BX:')
B = int(B)
C = raw_input('C:')
C = int(C)
#only calculate stuff under the root once
D = (B * B) - (4 * A * C)
if ( D < 0 ):
#negative root, not able to get with math.sqrt
print "Sorry mate, negative root. The math library is not a fan."
else:
#positive root(s)
x1 = (-B + math.sqrt(D) )/ (2 * A)
x2 = (-B - math.sqrt(D) )/ (2 * A)
print "root 1: ", x1
#check for repeated root
if(x1 != x2):
print "root 2: ", x2
<file_sep>/*
<NAME>
Fibonacci sequence generator
*/
#include <stdio.h> /*For output to console*/
#include <string.h> /*For checking flags*/
#include <stdlib.h> /*convert number to strings*/
#include <time.h> /*For measuring time*/
/*
TODO: writing to a file
Flags:
'-w' flag for writing to a file
'-t" flag for showing time taken to calculate
'-n #'flag for number limit, where # is the number
Current limit: unsigned long limit on laptop (64-bit) is around 9,700,000,000,000,000,000 in ANSI standard
Status: Works, no file write
*/
int main(int argc, char **argv){
/*Clock variables*/
clock_t start_t, end_t, total_t;
double totalTime;
/*Calculator variables*/
unsigned long a = 0;
unsigned long b = 1;
unsigned long c;
int i = 0;
unsigned long numberLimit = 9000000000000000000;
/*Flag variables*/
int temp;
int flags[2];
/*Checks flags*/
for(temp = 0; temp < argc; temp++){
/*write to file*/
if(strcmp(argv[temp],"-w")==0) flags[0] = 1;
/*Time the calculation*/
if(strcmp(argv[temp],"-t")==0) flags[1] = 1;
/*change number limit*/
if(strcmp(argv[temp],"-n")==0){
numberLimit = atoi(argv[temp+1]);
}
}
printf("Fibonacci Sequence generator\n");
if(flags[1]==1)start_t = clock();
/*Fibonacci Sequence Calculator*/
while(c < numberLimit || i < 101){
printf("%lu\n", c);
c = a + b;
a = b;
b = c;
++i;
}
if(flags[1]==1)end_t = clock();
/*Clock printouts*/
if(flags[1]==1){
total_t = (end_t - start_t);
printf("Total clocks to calculate: %li\n", total_t);
printf("clocks per second: %li\n", CLOCKS_PER_SEC);
totalTime = ((double) total_t / (double) CLOCKS_PER_SEC);
printf("Total time to calculate: %f seconds\n", totalTime);
}
return 0;
}
<file_sep>/* Sam (Sean) <NAME>
* Capitalize the first letter of every word in a sentence
* C (ansi / std=c90)
* Compiler: gcc (Ubuntu 7.3.0-27ubuntu1~18.04) 7.3.0
* February 18th, 2019
* */
#include <stdio.h>
#include <string.h>
#include <ctype.h>
int main(){
/*allocate space for 1024 characters. Dynamic memory allocation requires a lot more work.*/
char input[1024];
char *words;
char output[1024] = "";
/*prompt user imput*/
printf("Enter a string of words: ");
scanf("%[^\n]", input);
/*split string using space as a delimiter. other delimiters can be added if needed.*/
words = strtok(input, " ");
/*loop through each word*/
while(words != NULL){
/*turn the first letter into its uppercase form*/
words[0] = toupper(words[0]);
/* put resulting word into the ouput string*/
strcat(output, words);
strcat(output, " ");
words = strtok(NULL, " ");
}
/*print the resulting output*/
printf("%s\n", output);
return 0;
}
<file_sep># Small Projects
These are small projects I made in my (limited) free time.
C programming files can be compiled with the included makefile.
**C programming language:**
- bubblesort.c - A simple bubblesort algorithm
- fibonacci.c - A simple Fibonacci sequence generator
- prime.c - A simple prime number generator
- permute.c - A command line based word permutator
**Python 2 & 3 programming language:**
- quadraticPY2.py - Python 2 - A Simple quadratic root calculator
**Multiple (Multi) Languages**
Things written in multiple languages
- capitalize - Python, Java, C
| 357ba69bb946429c8f17f409f175e374a751fc5c | [
"Markdown",
"Makefile",
"Java",
"Python",
"C"
] | 10 | Java | Seangle/smallProjects | 53196fd206124538b96c0686818460692277c419 | 24077873ea65ba51d9024d584073d47856235e57 | |
refs/heads/master | <file_sep>// Constant used in the /data/config.json
// Versions
export const V_APP = 'app'
export const V_HUNTS = 'hunts'
export const V_SIDE_QUESTS = 'side-quests'
export const V_HUNTER_RANKS = 'hunter-ranks'<file_sep>import { SYSTEM_INIT, SYSTEM_CONFIG_RESPONSE, SYSTEM_VERSION_UPDATED } from '../constants/ActionTypes'
import { updateVersion, fetchHunts, fetchSideQuests } from '../actions/system'
import { V_APP, V_HUNTS, V_SIDE_QUESTS, V_HUNTER_RANKS } from '../constants/Config'
const initialState = {
// Start at 0, that way we can be sure to load a more recent one (in localstorage)
versions: {
[V_APP]: 0,
[V_HUNTER_RANKS]: 0,
[V_SIDE_QUESTS]: 0,
[V_HUNTS]: 0
},
loading: false,
error: undefined
}
const ACTIONS = {
[V_SIDE_QUESTS]: fetchSideQuests,
[V_HUNTS]: fetchHunts,
[V_APP]: (v) => updateVersion('app', v)
}
export default function system(state = initialState, action) {
switch(action.type) {
case SYSTEM_INIT:
return Object.assign({}, state, {loading: true})
case SYSTEM_CONFIG_RESPONSE:
if(action.success) {
const versions = action.json.versions
Object.keys(action.json.versions).forEach(key => {
if (versions[key] > state.versions[key]) {
if(ACTIONS[key] !== undefined) {
action.asyncDispatch(ACTIONS[key](versions[key]))
}
}
})
return Object.assign({}, state, {loading: false})
} else {
return Object.assign({}, state, {loading: false, error: action.err})
}
case SYSTEM_VERSION_UPDATED:
const versions = Object.assign({}, state.versions, { [action.key]: action.version })
console.log("SYSTEM_VERSION_UPDATED", action, versions)
return Object.assign({}, state, { versions })
default:
return state
}
}<file_sep>import React, { Component, PropTypes } from "react"
import { bindActionCreators } from 'redux'
import { connect } from 'react-redux'
import CompletableListView from '../components/CompletableListView'
import ListHeader from '../components/ListHeader'
import * as QuestsActions from '../actions/quests'
import * as NavActions from '../actions/navigation'
class QuestList extends Component {
static propTypes = {
items: PropTypes.array.isRequired,
filter: PropTypes.string.isRequired,
sort: PropTypes.string.isRequired,
actions: PropTypes.object.isRequired // TODO specify content of
}
handleQuestCompletion(id) {
this.props.actions.completeQuest(id)
}
render() {
const { filter, sort, items, nav, actions} = this.props
const completedItemsCount = items.reduce((count, item) =>
item.completed ? count + 1 : count,
0
)
const activeItemCount = items.length - completedItemsCount
return (
<div>
<ListHeader
subtitle="Quests"
onMenuClick={nav.openDrawer}
onShow={actions.filterQuests.bind(this)}
onSort={actions.sortQuests.bind(this)}
completedCount={completedItemsCount}
activeCount={activeItemCount}
activeFilter={filter}
activeSort={sort}
/>
<CompletableListView items={items}
onItemCompletion={this.handleQuestCompletion.bind(this)}
filter={filter}
sort={sort} />
</div>
)
}
}
function mapStateToProps(state) {
return state.quests
}
function mapDispatchToProps(dispatch) {
return {
actions: bindActionCreators(QuestsActions, dispatch),
nav: bindActionCreators(NavActions, dispatch)
}
}
export default connect(
mapStateToProps,
mapDispatchToProps
)(QuestList)
<file_sep>import React, { Component, PropTypes } from "react"
import { bindActionCreators } from 'redux'
import { connect } from 'react-redux'
import * as NavActions from '../actions/navigation'
import {List, ListItem} from 'material-ui/List';
import Subheader from 'material-ui/Subheader';
import Divider from 'material-ui/Divider';
import Checkbox from 'material-ui/Checkbox';
import Toggle from 'material-ui/Toggle';
import AppBar from 'material-ui/AppBar'
class Settings extends Component {
static propTypes = {
versions: PropTypes.object.isRequired,
openDrawer: PropTypes.func.isRequired
}
render() {
const { versions } = this.props
console.log(versions)
const versionsItem = Object.keys(versions).map((kind) => {
return (<ListItem
key={kind}
primaryText={kind}
disabled={true}
secondaryText={`Version ${versions[kind]}`}
/>)
})
return (
<div>
<header className="header">
<AppBar
title="FFXV Tracking - Settings"
onLeftIconButtonTouchTap={this.props.openDrawer} />
</header>
<List>
<Subheader>Versions</Subheader>
{versionsItem}
</List>
<Divider />
</div>
)
}
}
function mapStateToProps(state) {
return state.system
}
function mapDispatchToProps(dispatch) {
return {
openDrawer: bindActionCreators(NavActions, dispatch).openDrawer
}
}
export default connect(
mapStateToProps,
mapDispatchToProps
)(Settings)
<file_sep>import * as types from '../constants/ActionTypes';
// Complete toggle and Reset
export function completeHunt(id) {
return { type: types.COMPLETE_HUNT, id };
}
// UI
export function sortHunts(sort) {
return { type: types.HUNT_SORT, sort };
}
export function filterHunts(filter) {
return { type: types.HUNT_FILTER, filter };
}
// Network
export function requestHunts() {
return { type: types.REQUEST_HUNTS }
}
function slugify(text) {
return text.toString().toLowerCase()
.replace(/\s+/g, '-') // Replace spaces with -
.replace(/[^\w\-]+/g, '') // Remove all non-word chars
.replace(/\-\-+/g, '-') // Replace multiple - with single -
.replace(/^-+/, '') // Trim - from start of text
.replace(/-+$/, ''); // Trim - from end of text
}
export function receiveHunts(status, json, version) {
const action = { type: types.RESPONSE_HUNTS, status, receivedAt: Date.now(), version }
if(status === "success") {
return Object.assign(action, {hunts: json.map(e => Object.assign(e, {id: slugify(e.name)}))})
} else {
return Object.assign(action, {error: json})
}
}
<file_sep>import Perf from 'react-addons-perf'
import merge from 'lodash/merge'
import find from 'lodash/find'
import { COMPLETE_QUEST, QUEST_SORT, QUEST_FILTER, RESPONSE_SIDE_QUESTS } from '../constants/ActionTypes'
import { SHOW_ALL, SHOW_ACTIVE, SHOW_COMPLETED } from '../constants/Filters'
import { SORT_NAME, SORT_REGION, SORT_LEVEL, SORT_LOCATION } from '../constants/Filters'
import { V_SIDE_QUESTS } from '../constants/Config'
import { updateVersion } from '../actions/system'
// TODO Have an object with {quests, filter, sort}
const initialState = {
items: [],
filter: SHOW_ALL,
sort: SORT_NAME
};
let lastLocPayload = {pathname: 'none'}
export default function quests(state = initialState, action) {
switch (action.type) {
case RESPONSE_SIDE_QUESTS:
if(action.status === 'success') {
const items = action.quests.map((item) => {
return merge({}, find(state.items, {'id': item.id}), item)
})
action.asyncDispatch(updateVersion(V_SIDE_QUESTS, action.version))
return Object.assign({}, state, { items, error: undefined });
} else {
return Object.assign({}, state, {error: action.error});
}
case COMPLETE_QUEST:
const completedQuests = state.items.map(quest =>
quest.id === action.id
? Object.assign({}, quest, { completed: !quest.completed })
: quest
);
return Object.assign({}, state, {items: completedQuests});
case QUEST_FILTER:
return Object.assign({}, state, {filter: action.filter})
case QUEST_SORT:
return Object.assign({}, state, {sort: action.sort})
case '@@router/LOCATION_CHANGE':
/*
const locPayload = action.payload
console.log(`Location changed. Printing performance from ${lastLocPayload.pathname} to ${locPayload.pathname}.`)
Perf.stop()
const measurements = Perf.getLastMeasurements()
Perf.printInclusive(measurements)
Perf.printExclusive(measurements)
Perf.printWasted(measurements)
console.log(`Collecting performance from ${locPayload.pathname} to next location change.`)
Perf.start()
*/
default:
return state;
}
}
<file_sep>import React, { Component, PropTypes } from "react"
import { bindActionCreators } from 'redux'
import { connect } from 'react-redux'
import CompletableListView from '../components/CompletableListView'
import ListHeader from '../components/ListHeader'
import * as HuntsActions from '../actions/hunts'
import * as NavActions from '../actions/navigation'
class HuntList extends Component {
static propTypes = {
items: PropTypes.array.isRequired,
filter: PropTypes.string.isRequired,
sort: PropTypes.string.isRequired,
actions: PropTypes.object.isRequired // TODO specify content of
}
handleHuntCompletion(id) {
this.props.actions.completeHunt(id)
}
render() {
const { filter, sort, items, nav, actions } = this.props
const completedItemsCount = items.reduce((count, item) =>
item.completed ? count + 1 : count,
0
)
const activeItemCount = items.length - completedItemsCount
return (
<div>
<ListHeader
subtitle="Hunts"
onMenuClick={nav.openDrawer}
onShow={actions.filterHunts.bind(this)}
onSort={actions.sortHunts.bind(this)}
completedCount={completedItemsCount}
activeCount={activeItemCount}
activeFilter={filter}
activeSort={sort}
/>
<CompletableListView items={items}
onItemCompletion={this.handleHuntCompletion.bind(this)}
filter={filter}
sort={sort} />
</div>
)
}
}
function mapStateToProps(state) {
return state.hunts
}
function mapDispatchToProps(dispatch) {
return {
actions: bindActionCreators(HuntsActions, dispatch),
nav: bindActionCreators(NavActions, dispatch)
}
}
export default connect(
mapStateToProps,
mapDispatchToProps
)(HuntList)
<file_sep>import { routerMiddleware } from 'react-router-redux'
import { hashHistory } from 'react-router'
import thunkMiddleware from 'redux-thunk'
import asyncDispatchMiddleware from './asyncDispatchMiddleware'
export const router = routerMiddleware(hashHistory)
export const thunk = thunkMiddleware
export const asyncDispatch = asyncDispatchMiddleware<file_sep>import * as types from '../constants/ActionTypes';
// Complete toggle and Reset
export function completeQuest(id) {
return { type: types.COMPLETE_QUEST, id };
}
// UI
export function sortQuests(sort) {
return { type: types.QUEST_SORT, sort };
}
export function filterQuests(filter) {
return { type: types.QUEST_FILTER, filter };
}
// Network
export function requestSideQuests() {
return { type: types.REQUEST_SIDE_QUESTS }
}
function slugify(text) {
return text.toString().toLowerCase()
.replace(/\s+/g, '-') // Replace spaces with -
.replace(/[^\w\-]+/g, '') // Remove all non-word chars
.replace(/\-\-+/g, '-') // Replace multiple - with single -
.replace(/^-+/, '') // Trim - from start of text
.replace(/-+$/, ''); // Trim - from end of text
}
export const receiveSideQuests = (status, json, version) => (dispatch) => {
const action = { type: types.RESPONSE_SIDE_QUESTS, status, receivedAt: Date.now(), version }
if(status === "success") {
dispatch(Object.assign(action, {quests: json.map(e => Object.assign(e, {id: slugify(e.name)}))}))
} else {
dispatch(Object.assign(action, {error: json}))
}
}
<file_sep>import React, { PropTypes, Component } from 'react'
import IconMenu from 'material-ui/IconMenu'
import IconButton from 'material-ui/IconButton'
import FontIcon from 'material-ui/FontIcon'
import MenuItem from 'material-ui/MenuItem'
import DropDownMenu from 'material-ui/DropDownMenu'
import { Toolbar, ToolbarGroup, ToolbarSeparator, ToolbarTitle } from 'material-ui/Toolbar'
import InboxIcon from 'material-ui/svg-icons/content/inbox'
import LoopIcon from 'material-ui/svg-icons/av/loop'
import SortByAlphaIcon from 'material-ui/svg-icons/av/sort-by-alpha'
import ArchiveIcon from 'material-ui/svg-icons/content/archive'
import FilterIcon from 'material-ui/svg-icons/content/filter-list'
import SortIcon from 'material-ui/svg-icons/content/sort'
import AppBar from 'material-ui/AppBar'
import { SHOW_ALL, SHOW_COMPLETED, SHOW_ACTIVE } from '../constants/Filters'
import { SORT_NAME, SORT_LEVEL, SORT_REGION, SORT_LOCATION } from '../constants/Filters'
const defaultStyle = {
marginLeft: 20
}
const FILTER_TITLES = {
[SHOW_ALL]: 'All',
[SHOW_ACTIVE]: 'Active',
[SHOW_COMPLETED]: 'Completed'
}
const FILTER_ICONS = {
[SHOW_ALL]: <InboxIcon />,
[SHOW_ACTIVE]: <LoopIcon />,
[SHOW_COMPLETED]: <ArchiveIcon />
}
const SORT_TITLES = {
[SORT_NAME]: 'By Name',
[SORT_LEVEL]: 'By Level',
[SORT_REGION]: 'By Region',
[SORT_LOCATION]: 'By Location'
}
const capitalize = (s) => s.charAt(0).toUpperCase() + s.slice(1)
class Header extends Component {
static propTypes = {
onMenuClick: PropTypes.func.isRequired,
subtitle: PropTypes.string.isRequired,
onSort: PropTypes.func.isRequired,
onShow: PropTypes.func.isRequired,
completedCount: PropTypes.number.isRequired,
activeCount: PropTypes.number.isRequired,
activeFilter: PropTypes.string.isRequired,
activeSort: PropTypes.string.isRequired
}
countForFilter(filter) {
const { activeCount, completedCount } = this.props
if (filter === SHOW_ALL) return activeCount + completedCount
if (filter === SHOW_ACTIVE) return activeCount
if (filter === SHOW_COMPLETED) return completedCount
}
renderFilterLink(filter) {
const { onShow, activeFilter } = this.props
const count = this.countForFilter(filter)
const title = FILTER_TITLES[filter] + (count > 0 ? ' (' + count + ')' : '')
const style = (activeFilter === filter) ? {fontWeight: 'bold'} : {}
return (
<MenuItem key={filter}
style={style}
focusState={'keyboard-focused'}
primaryText={`Show ${title}`}
leftIcon={FILTER_ICONS[filter]}
onTouchTap={() => onShow(filter)}
/>
)
}
renderSortLink(sort) {
const { onSort, activeSort } = this.props
const style = (activeSort === sort) ? {fontWeight: 'bold'} : {}
return (
<MenuItem key={sort} style={style} primaryText={SORT_TITLES[sort]} onTouchTap={() => onSort(sort)} />
)
}
render() {
const {onSort, onShow, subtitle} = this.props
const sortButton = (
<IconButton touch={true}>
<SortIcon />
</IconButton>
)
const moreButton = (
<IconButton touch={true}>
<FilterIcon />
</IconButton>
)
return (
<header className="header">
<AppBar title={`FFXV Tracking - ${capitalize(subtitle)}`} onLeftIconButtonTouchTap={this.props.onMenuClick}>
<ToolbarGroup>
<IconMenu iconButtonElement={sortButton}>
{[SORT_NAME, SORT_LEVEL, SORT_REGION, SORT_LOCATION].map(sort =>
this.renderSortLink(sort)
)}
</IconMenu>
<IconMenu iconButtonElement={moreButton} >
{[SHOW_ALL, SHOW_ACTIVE, SHOW_COMPLETED].map(filter =>
this.renderFilterLink(filter)
)}
</IconMenu>
</ToolbarGroup>
</AppBar>
</header>
)
}
}
export default Header
<file_sep>import { createStore, applyMiddleware, compose } from 'redux';
import { routerMiddleware } from 'react-router-redux'
import { hashHistory } from 'react-router'
import persistState from 'redux-localstorage'
import rootReducer from '../reducers';
import * as middlewares from '../middleware';
export default function configureStore(initialState) {
const composeEnhancers = window.__REDUX_DEVTOOLS_EXTENSION_COMPOSE__ || compose;
const store = createStore(
rootReducer,
initialState,
composeEnhancers(
applyMiddleware(...Object.values(middlewares)),
persistState()
)
);
if (module.hot) {
// Enable Webpack hot module replacement for reducers
module.hot.accept('../reducers', () => {
const nextReducer = require('../reducers');
store.replaceReducer(nextReducer);
});
}
return store;
}
<file_sep>export const SHOW_ALL = 'show_all'
export const SHOW_COMPLETED = 'show_completed'
export const SHOW_ACTIVE = 'show_active'
export const SORT_NAME = 'order_name'
export const SORT_LEVEL = 'order_level'
export const SORT_REGION = 'order_chapter'
export const SORT_LOCATION = 'order_location'<file_sep>import { combineReducers } from 'redux'
import { routerReducer } from 'react-router-redux'
import system from './system'
import quests from './quests'
import drawer from './drawer'
import hunts from './hunts'
const rootReducer = combineReducers({
system,
quests,
drawer,
hunts,
routing: routerReducer
})
export default rootReducer
<file_sep>const fs = require('fs')
const config = require('../data/config.json')
const merge = require('lodash/merge')
const newAppVersion = config.versions.app + 1
const newConfig = merge(config, {
versions: {
app: newAppVersion
}
})
fs.writeFileSync('./data/config.json', JSON.stringify(newConfig))
fs.writeFileSync('./VERSION', newAppVersion)<file_sep>const fs = require('fs')
const cheerio = require('cheerio')
const _ = require('lodash')
// Selector to the main section
const ghContentSelector = 'body > div.shell.container-content-main > div.gh-shell > div.container_24.clear > div.grid_16.gh-content > div.grid_12.push_4.alpha.omega.bodyCopy.gh-content'
function getRewards(td) {
return td.contents()
.filter(function(){
return this.nodeType == 3
})
.map(function(){
return this.data
})
.toArray()
.map((e) => e.trim().replace(',', ''))
.filter((s) => s.length > 0)
}
function parseIGNWikiPage(file, regionSuffixToReplace, parseRow, arrToQuestObject) {
const $ = cheerio.load(file)
const contentNode = $(ghContentSelector)
return $('h3.gh-sectionHeader', contentNode).toArray().map((element, index) => {
const e = $(element)
const region = e.contents()
.filter(function () {
return !(this.name === "a" && this.attribs.hasOwnProperty('class'))
}).text()
.replace(regionSuffixToReplace + 's', '')
.replace(regionSuffixToReplace, '')
const table = e.next().next()
const data = $('tr', table).toArray()
.map((rawTr, i) => {
// Ignore Headers
if(i === 0) {
return undefined
}
const tr = $(rawTr)
const tds = $('td', tr).toArray()
return parseRow($, tds)
})
.filter((e) => e != undefined)
.map(arrToQuestObject)
return {region, data}
}).reduce((acc, val) => {
return acc.concat(val.data.map((e) => Object.assign(e, {region: val.region})))
}, [])
}
//
// Side Quests functions
//
function parseQuestRow($, tds) {
const isCid = tds.length === 2
return tds.map((rawTd, i) => {
if(isCid) {
if(i == 0) {
return [
$(rawTd).text().trim(),
_.find(rawTd.children, (c) => c.name === 'a').attribs.href
]
} else if(i == 1) {
return getRewards($(rawTd))
} else {
return $(rawTd).text().trim()
}
} else {
if(i == 0) {
const a = _.find(rawTd.children, (c) => c.name === 'a')
return [
$(rawTd).text().trim(),
(a != undefined) ? a.attribs.href : undefined
]
} else if(i == 3) {
const td = $(rawTd)
return getRewards(td)
} else {
return $(rawTd).text().trim()
}
}
})
}
// TODO Match 'Chapter' and 'Ch'
const chapterRegex = /(.*)\(Chapter\ (\d{1,2})\)/
function arrToQuestObject(arr) {
// Cid
if(arr.length === 2) {
return {
name: arr[0][0],
link: arr[0][1],
rewards: arr[1]
}
}
// Side quests
const result = chapterRegex.exec(arr[2])
let location, chapter
if(result === null || result.length !== 3) {
location = arr[2]
} else {
location = result[1]
chapter = parseInt(result[2], 10)
}
return {
name: arr[0][0],
link: arr[0][1],
level: parseInt(arr[1], 10),
chapter, location,
rewards: arr[3]
}
}
//
// Hunts functions
//
function parseHuntsRow($, tds) {
const isAltissia = tds.length === 5;
return tds.map((rawTd, i) => {
if(isAltissia) {
if(i === 4) {
return getRewards($(rawTd))
} else {
return $(rawTd).text().trim()
}
} else {
if(i === 3 || i === 5) {
return getRewards($(rawTd))
} else {
return $(rawTd).text().trim()
}
}
})
}
function arrToHuntObject(arr) {
// Altissia
if(arr.length === 5) {
return {
name: arr[0],
level: parseInt(arr[1], 10),
location: arr[2],
enemy: arr[3],
rewards: arr[4]
}
}
// Regular hunts
// TODO Regex on habitat to extract time
// TODO Regex on each enemy to extract number
// TODO Regex on Location for (region, location, hunter rank)
return {
name: arr[0],
level: arr[1],
location: arr[2],
enemy: arr[3],
habitat: arr[4],
rewards: arr[5]
}
}
//
// Effectively parsing files
//
const sideQuests = parseIGNWikiPage(
fs.readFileSync('./fixtures/side-quests.html').toString(),
' Side Quest', parseQuestRow, arrToQuestObject
)
const hunts = parseIGNWikiPage(
fs.readFileSync('./fixtures/bounty-hunts.html').toString(),
' Bounty Hunts', parseHuntsRow, arrToHuntObject
)
fs.writeFileSync('./data/side-quests.json', JSON.stringify(sideQuests))
fs.writeFileSync('./data/hunts.json', JSON.stringify(hunts))<file_sep>import {OPEN_DRAWER, CLOSE_DRAWER, OPEN_PAGE} from '../constants/ActionTypes';
const initialState = {
open: false
};
export default function drawer(state = initialState, action) {
switch(action.type) {
case OPEN_DRAWER:
return {
open: true
};
case CLOSE_DRAWER:
case OPEN_PAGE:
return {
open: false
};
default:
return state;
}
}<file_sep>// Side Quests
export const COMPLETE_QUEST = 'COMPLETE_QUEST'
export const QUEST_SORT = 'QUEST_SORT'
export const QUEST_FILTER = 'QUEST_FILTER'
export const REQUEST_SIDE_QUESTS = 'REQUEST_SIDE_QUESTS'
export const RESPONSE_SIDE_QUESTS = 'RESPONSE_SIDE_QUESTS'
// Hunts
export const COMPLETE_HUNT = 'COMPLETE_HUNT'
export const HUNT_SORT = 'HUNT_SORT'
export const HUNT_FILTER = 'HUNT_FILTER'
export const REQUEST_HUNTS = 'REQUEST_HUNTS'
export const RESPONSE_HUNTS = 'RESPONSE_HUNTS'
// Drawer
export const OPEN_DRAWER = 'OPEN_DRAWER'
export const CLOSE_DRAWER = 'CLOSE_DRAWER'
// Navigation
export const OPEN_PAGE = 'OPEN_PAGE'
// Initialisation
export const SYSTEM_INIT = 'SYSTEM_INIT'
export const SYSTEM_CONFIG_RESPONSE = 'SYSTEM_CONFIG_RESPONSE'
export const SYSTEM_VERSION_UPDATED = 'SYSTEM_VERSION_UPDATED' | 5bec04446e7178fb60b7f336ebd2db8485a1c87e | [
"JavaScript"
] | 17 | JavaScript | fmonniot/ffxv-tracker | 546ff91c417707761a86b34db48e11d8aa2ddf83 | 0fd78958c3c0e53bc499c39a8aa81dac2b33e59a | |
refs/heads/master | <repo_name>louiemd/altwebservices<file_sep>/functions.php
<?php
// This theme uses wp_nav_menu() in one location.
register_nav_menu( 'primary', __( 'Primary Menu', 'main-nav' ) );
function astro_add_dropdown_class($classes, $item) {
global $wpdb;
$has_children = $wpdb->get_var("
SELECT COUNT(meta_id)
FROM wp_postmeta
WHERE meta_key='_menu_item_menu_item_parent'
AND meta_value='".$item->ID."'
");
// add the class dropdown to the current list
if ($has_children > 0) array_push($classes,'dropdown');
return $classes;
}
add_filter( 'nav_menu_css_class', 'astro_add_dropdown_class', 10, 2);
if ( function_exists('register_sidebar') ) {
register_sidebar(array(
'before_widget' => '<div id="%1$s" class="widget well %2$s">',
'after_widget' => '</div>',
'before_title' => '<h4 class="widgettitle"><em>',
'after_title' => '</em></h4>',
));
}
// Post thumbnail
if ( function_exists( 'add_theme_support' ) ) {
add_theme_support( 'post-thumbnails' );
set_post_thumbnail_size( 118, 118, true ); // default Post Thumbnail dimensions (cropped)
}<file_sep>/header.php
<!DOCTYPE html>
<html>
<head>
<title>
<?php if (function_exists('is_tag') && is_tag()) {
single_tag_title('Tag Archive for "'); echo '" - ';
} elseif (is_archive()) {
wp_title(''); echo ' Archive - ';
} elseif (is_search()) {
echo 'Search for "'.wp_specialchars($s).'" - ';
} elseif (!(is_404()) && (is_single()) || (is_page())) {
wp_title(''); echo ' - ';
} elseif (is_404()) {
echo 'Not Found - ';
}
if (is_home()) {
bloginfo('name'); echo ' - '; bloginfo('description');
} else {
bloginfo('name');
}
if ($paged > 1) {
echo ' - page '. $paged;
} ?>
</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Bootstrap -->
<link href="<?php bloginfo('stylesheet_directory'); ?>/css/bootstrap.min.css" rel="stylesheet" media="screen">
<link href="<?php bloginfo('stylesheet_directory'); ?>/css/bootstrap-responsive.css" rel="stylesheet">
<link rel="stylesheet" type="text/css" media="screen" href="<?php bloginfo( 'stylesheet_url' ); ?>" />
<?php wp_head(); ?>
</head>
<body <?php body_class(); ?>>
<div id="header">
<div class="container">
<h1 class="pull-left"><a href="<?php echo get_option('home'); ?>/"><img src="<?php bloginfo('stylesheet_directory'); ?>/images/logo2.png" alt="<?php bloginfo('name'); ?>"></a></h1>
<?php
wp_nav_menu( array(
'menu' => 'Primary Menu',
'theme_location' => '',
'container' =>false,
'menu_class' => '',
'echo' => true,
'before' => '',
'after' => '',
'link_before' => '',
'link_after' => '',
'depth' => 0,
'items_wrap' => '<ul class="nav nav-pills pull-right">%3$s</ul>'
));
?>
</div>
</div> <!-- end header -->
<?php if(is_front_page()) { ?>
<!-- Carousel
================================================== -->
<div id="myCarousel" class="carousel slide">
<div class="container">
<div class="carousel-inner">
<div class="item active">
<img src="<?php bloginfo('stylesheet_directory'); ?>/images/altweb-slider-1.png" alt= "" />
</div> <!-- item -->
<div class="item">
<img src="<?php bloginfo('stylesheet_directory'); ?>/images/altwebSEOSlide2.png" alt= "" />
</div> <!-- item -->
<div class="item">
<img src="<?php bloginfo('stylesheet_directory'); ?>/images/altwebWebDevSlide2.png" alt= "" />
</div> <!-- item -->
</div> <!-- carousel-inner -->
<a class="left carousel-control" href="#myCarousel" data-slide="prev">‹</a>
<a class="right carousel-control" href="#myCarousel" data-slide="next">›</a>
</div>
</div><!-- /.carousel -->
<?php } elseif (is_404()) { ?>
<div id="masthead">
<div class="container">
<h1>404 Page</h1>
</div>
</div>
<?php } else { ?>
<div id="masthead">
<div class="container">
<h1><?php the_title(); ?></h1>
</div>
</div>
<?php } ?><file_sep>/page.php
<?php get_header(); ?>
<!-- Example row of columns -->
<div id="contentpart">
<div class="container">
<div class="row-fluid">
<div class="span8">
<?php if(is_front_page()): ?>
<h2 style="margin-bottom:30px"><em><?php the_title(); ?></em></h2>
<?php endif; ?>
<?php if (have_posts()) : while (have_posts()) : the_post(); ?>
<?php the_content(); ?>
<?php endwhile; endif; ?>
</div>
<div class="span4">
<?php if ( !function_exists('dynamic_sidebar') || !dynamic_sidebar() ) : ?><?php endif; ?>
</div>
</div> <!-- row-fluid -->
</div> <!-- container -->
</div> <!-- contentpart -->
<?php get_footer(); ?><file_sep>/single.php
<?php get_header(); ?>
<!-- Example row of columns -->
<div id="contentpart">
<div class="container">
<div class="row-fluid">
<div class="span8">
<?php if (have_posts()) : while (have_posts()) : the_post(); ?>
<h2 class="bottomElement"><?php the_title(); ?></h2>
<p class="meta">
<em><?php the_date(); ?> by <?php the_author() ?></em>
</p>
<?php the_content(); ?>
<?php comments_template(); ?>
<?php endwhile; else: ?>
<p>Sorry, no posts matched your criteria.</p>
<?php endif; ?>
</div>
<div class="span4">
<?php if ( !function_exists('dynamic_sidebar') || !dynamic_sidebar() ) : ?><?php endif; ?>
</div>
</div> <!-- row-fluid -->
</div> <!-- container -->
</div> <!-- contentpart -->
<?php get_footer(); ?><file_sep>/footer.php
<div class="subfooter">
<div class="container">
<div class="row-fluid">
<div class="span4" style="margin-left:0">
<h4>Recent Works</h4>
<div style="margin-left:0" class="span5">
<div class="thumbnail">
<img style="width: 119px;" data-src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/holder/holder.js/300x200" src="<?php bloginfo('stylesheet_directory'); ?>/images/thumb1.png" alt="">
</div>
</div>
<div class="span5">
<div class="thumbnail">
<img style="width: 119px;" data-src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/holder/holder.js/300x200" src="<?php bloginfo('stylesheet_directory'); ?>/images/thumb1.png" alt="">
</div>
</div>
<div class="clearfix"></div>
<p></p>
<div style="margin-left:0" class="span5">
<div class="thumbnail">
<img style="width: 119px;" data-src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/holder/holder.js/300x200" src="<?php bloginfo('stylesheet_directory'); ?>/images/thumb1.png" alt="">
</div>
</div>
<div class="span5">
<div class="thumbnail">
<img style="width: 119px;" data-src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/holder/holder.js/300x200" src="<?php bloginfo('stylesheet_directory'); ?>/images/thumb1.png" alt="">
</div>
</div>
<div class="clearfix"></div>
<h3><a href="#" class="btn btn-info btn-medium">View our portfolio »</a></h3>
<!-- <h3>Champ Soft</h3>
<div class="thumbnail">
<img style="width: 297px; height: 180px;" data-src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/holder/holder.js/300x200" src="<?php bloginfo('stylesheet_directory'); ?>/images/thumb1.png" alt="">
</div>
<p>Some descriptions here</p>
<p><a class="btn" href="#">View details »</a></p> -->
</div>
<div class="span4">
<h4>Newsletter</h4>
<form>
<fieldset>
<ul class="cleanform">
<li><label>Name:</label></li>
<li><input type="text"></li>
<li><label>Email:</label></li>
<li><input type="text"></li>
<li><button type="submit" class="btn">Submit</button></li>
</ul>
</fieldset>
</form>
</div>
<div class="span4">
<h4>Reach Us</h4>
<p><i class="icon-envelope"></i> <a href="mailto:<EMAIL>"><EMAIL></a></p>
</div>
</div>
<!-- / row-fluid -->
</div>
<!-- /container -->
</div>
<!-- subfooter -->
<div class="footer">
<div class="container">
<p class="pull-left">© <?php echo date('Y'); ?> Copyright <?php bloginfo('name'); ?></p>
<p class="pull-right">
<a href="#">Facebook</a> | <a href="#">Twitter</a>
</p>
<div class="clearfix"></div>
</div>
</div>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/jquery.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap.min.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap-transition.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap-alert.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap-modal.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap-dropdown.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap-scrollspy.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap-tab.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap-tooltip.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap-popover.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap-button.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap-collapse.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap-carousel.js"></script>
<script src="<?php bloginfo('stylesheet_directory'); ?>/assets/js/bootstrap-typeahead.js"></script>
<script>
$('.dropdown > a').append('<b class="caret"></b>').dropdown();
$('.dropdown .sub-menu').addClass('dropdown-menu');
</script>
<?php wp_footer(); ?>
</body>
</html><file_sep>/index.php
<?php get_header(); ?>
<!-- Example row of columns -->
<div id="contentpart">
<div class="container">
<div class="row-fluid">
<div class="span8">
<?php if (have_posts()) : ?>
<?php while (have_posts()) : the_post(); ?>
<div style="margin-bottom:30px" class="post">
<?php //the_post_thumbnail(array('class' => 'alignleft')); ?>
<div class="pull-left">
<h2><a href="<?php the_permalink() ?>"><?php the_title(); ?></a></h2>
<p class="meta">
<span class="label label-inverse"><?php the_date(); ?> by <?php the_author() ?></span>
</p>
</div>
<div class="commentBubble pull-right" style="padding-top:25px;">
Comments <span class="badge badge-info"><a style="color:#fff; font-size:18px;" href="<?php comments_link(); ?>"><?php comments_number( '0','1','%'); ?></a></span>
</div>
<div class="clearfix"></div>
<?php the_excerpt(); ?>
<a class="btn" href="<?php the_permalink() ?>">… Read More</a>
</div> <!-- end post-->
<?php endwhile; ?>
<div class="navigation">
<div class="alignleft"><?php next_posts_link('« Older Entries') ?></div>
<div class="alignright"><?php previous_posts_link('Newer Entries »') ?></div>
</div>
<?php else : ?>
<h2 class="center">Not Found</h2>
<p class="center">Sorry, but you are looking for something that isn't here.</p>
<?php endif; ?>
</div>
<div class="span4">
<?php if ( !function_exists('dynamic_sidebar') || !dynamic_sidebar() ) : ?><?php endif; ?>
</div>
</div> <!-- row-fluid -->
</div> <!-- container -->
</div> <!-- contentpart -->
<?php get_footer(); ?><file_sep>/404.php
<?php get_header(); ?>
<!-- Example row of columns -->
<div id="contentpart">
<div class="container">
<div class="row-fluid">
<div class="span8">
<p>Seems you are lost. Here let me show you way <a href="/">out.</a></p>
</div>
<div class="span4">
<?php if ( !function_exists('dynamic_sidebar') || !dynamic_sidebar() ) : ?><?php endif; ?>
</div>
</div> <!-- row-fluid -->
</div> <!-- container -->
</div> <!-- contentpart -->
<?php get_footer(); ?> | 41fa7209d65e1379a0346a8a25e5dfc8f622fd2d | [
"PHP"
] | 7 | PHP | louiemd/altwebservices | 9cff0fcacbe4243aa52a4fc5c66217ab92b760bf | a90859de5bd47a239546ede587f05f460890efd3 | |
refs/heads/master | <repo_name>kaizhang32/CSSAtechWeb<file_sep>/kai.py
class User:
def __init__(self, username, password, info, author):
self.username = username
self.password = <PASSWORD>
self.info = info
author="%s,%s,%s"%("lizhi","caoyudong","zhangzhihang")
self.author = author
def show_info(self):
userinfo = "用户名:%s\n" % self.username
userinfo = "密码:%s\n" % self.password
userinfo = "个人信息:%s" %self.info
print(userinfo)
def edit_info(self, info):
self.info = info
def change_pwd(self,password):
self.password = "<PASSWORD>"
pwd = input("please enter current password:")
if pwd == self.password:
print("sucessful,please enter new pasword:")
self.password=password
def add_author(self,add_str):
add_str="%s"%(",fangyue")
self.author += add_str[0:8]
def reduce_author(self):
part1_author=self.author[0:17]
part2_author=self.author[30:]
self.author = part1_author + part2_author
def retuen_username(self,username):
self.username = username
return
class Activity:
def __init__(self, title, content, date, member_num=0):
self.title = title
self.content = content
self.date = date
self.member_num = str(member_num)
def add_member(self):
self.member_num = str(int(self.member_num)+1)
def reduce_member(self):
self.member_num = str(int(self.member_num)-1)
def edit_content(self, content):
self.content = content
def lst_function():
lst = list()
for i in range(1, 6):
lst.append(i)
for i in range(6, 11):
lst.append(i)
lst.remove(4)
print(lst)
def dict_function():
dic = dict()
dic['Li'] = 10411234
print(dic.get('Li'))
print(dic.get('Lee', 0))
def onetoten_function():
onetoten = list()
for i in range(1,10):
onetoten.append(i)
print(onetoten)
def reduce1to10_function():
from .onetoten import reduce1to10
i=len(reduce1to10)
if i>0:
reduce1to10.pop(i)
i-=1
else:
print("list is empty")
<file_sep>/README.md
<<<<<<< HEAD
# CSSAwebKai
CSSA web Python
=======
# CSSAtechWeb
>>>>>>> 5f20385bb7822561c8df27d5af42b45b93e8de51
<file_sep>/P5Danni Wang.py
# -*- coding: utf-8 -*-
"""
Created on Sun Oct 1 19:33:37 2017
@author: Danni
"""
def plural(word):
if word[-2:] in ['ay','ey','iy','oy','uy']:
return word+ 's'
elif word[-2:] in ['cy','by','dy','fy','gy','hy','jy','qy','ky','ly','my','ny','py','ry','sy','ty','vy','wy','xy','yy','zy']:
return word[:-1] + 'ies'
elif word[-1] in 'sxoz' or word[-2:] in ['sh', 'ch']:
return word + 'es'
else:
return word + 's'
t=input('Enter a sentence:')
l=t.split()
a=" ".join([plural(word) for word in l])
plural(a)
print(a)<file_sep>/P4Danni.py
# -*- coding: utf-8 -*-
"""
Created on Sun Sep 24 15:36:47 2017
@author: Danni
"""
import random
def judge(guess):
gue = int(guess)
if (gue<0) or (gue>20):
print('Wrong,Please input a number between 0 to 20.')
guess = input('Please input a number between 0 to 20:')
judge(guess)
return gue
def istrue(n,guess):
n=random.randint(1,21)
print(n)
b=str(input('Hello! What is your name?'))
print('Well,',b,',I am thinking of a number between 1 and 20.')
guess=int(input( 'Take a guess:'))
guess = judge(guess)
for a in range(1,6):
if n!= guess:
if guess<n:
print('Your guess is too low.')
guess=int(input('Take a guess:'))
guess = judge(guess)
elif guess>n:
print('guess is high.')
guess=int(input('Take a guess:'))
guess = judge(guess)
if n == guess:
print('Good Job,',b,',You have guessed my number in',a+1,'guesses!')
#guessed = True
break
if a == 5:
print('The number I was thinking of was',n)
return;
istrue(n,guess)
#break
#if a==6:
#print('The number I was thinking of was',n)
<file_sep>/week1.py
#first question
class user()
def _init_(self,user_name,user_pin,user_profile):
self.name=user_name
self.pin=user_pin
self.profile=user_profile
def default(self):
self.name="zjzk99"
self.pin="a2358686b"
self.profile="male"
def custom(self,user_profile):
self.profile=user_profile
#second question
class activity()
def _init_(self,title,matter,data,num):
self.title=title
self.matter=matter
self.data=data
self.num=num
def add_num(self,plus):
self.num=num+"%s" % plus
def reduce_num(self,minus):
self.num=num-"%s" % minus
def custom(self,matter):
self.matter=matter
#third question
list=[1,2,3,4,5]
for item in range(6,11):
list.append(item)
list.remove(4)
print(list)
#forth question
dic={key:"Kai_Zhang",
value:"10430213"
}
print "key: %s" % dic.get("key")
print "value: %s" % dic.get("value")
<file_sep>/week1answer.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Sun Sep 17 11:48:17 2017
@author: jackylee
"""
class User:
def __init__(self, username, password, info):
self.username = username
self.password = <PASSWORD>
self.info = info
def show_info(self):
userinfo = "用户名:%s\n" % self.username
userinfo += "密码:<PASSWORD>" % self.password
userinfo += "个人信息:%s" %self.info
print(userinfo)
def edit_info(self, info):
self.info = info
class Activity:
# member_num 这个参数可以设置默认
def __init__(self, title, content, date, member_num=0):
self.title = title
self.content = content
self.date = date
self.member_num = str(member_num)
def add_member(self):
self.member_num = str(int(self.member_num)+1)
'''
也可以写成如下,方便理解:
number = int(self.member_num) + 1
self.member_num = str(number)
'''
def reduce_member(self):
self.member_num = str(int(self.member_num)-1)
'''
也可以写成如下,方便理解:
number = int(self.member_num) - 1
self.member_num = str(number)
'''
def edit_content(self, content):
self.content = content
def lst_function():
lst = list()
# for循环要会写
for i in range(1, 6):
lst.append(i)
for i in range(6, 11):
lst.append(i)
lst.remove(4)
print(lst)
def dict_function():
dic = dict()
dic['Li'] = 10411234
# 学会使用dict.get()方法
print(dic.get('Li'))
# 如果不存在的话,这样做就不会报错
print(dic.get('Lee', 0))
<file_sep>/assign2.py
print('hello! python for everyone!!')
<file_sep>/P5Danni.py
# -*- coding: utf-8 -*-
"""
Created on Sun Oct 1 18:48:03 2017
@author: Kai
"""
def plural(word):
if word.endswith('y'):
return word[:-1] + 'ies'
elif word[-1] in 'sx' or word[-2:] in ['sh', 'ch']:
return word + 'es'
elif word.endswith('an'):
return word[:-2] + 'en'
else:
return word + 's'
t=input('Enter a sentence:')
l=t.split()
a=" ".join([plural(word) for word in l])
plural(a)
print(a)
| c0e60cd26821c771310b55c3c844c301d3337860 | [
"Markdown",
"Python"
] | 8 | Python | kaizhang32/CSSAtechWeb | 56510bf9fbaaa10001819fb48d9654cfb650b96b | ee392fedc3567b1cb625c4eb7704183bab490c57 | |
refs/heads/master | <repo_name>hjl12/DDup<file_sep>/src/cn/mumu/architecture/model/future/success/MyFutureData.java
package cn.mumu.architecture.model.future.success;
import java.util.concurrent.locks.LockSupport;
public class MyFutureData implements MyData {
private MyRealData realData;
private boolean isRealData = false;
// @Override
// public synchronized int getResult() {
// if (!isRealData) {
// try {
// System.out.println("线程还没有计算好,wait()");
// wait();
// } catch (InterruptedException e) {
// // TODO Auto-generated catch block
// e.printStackTrace();
// }
// }
// return realData.getResult();
// }
//
// public synchronized void setRequest(MyRealData my) {
// if (!isRealData) {
// System.out.println("计算完成设置realdata到futuredata");
// this.realData = my;
// isRealData = true;
// notifyAll();
// } else {
// return;
// }
//
// }
@Override
public int getResult() {
if (!isRealData) {
System.out.println("线程还没有计算好,wait()");
}
LockSupport.park();
return realData.getResult();
}
public void setRequest(MyRealData my,Thread t ) {
if (!isRealData) {
System.out.println("计算完成设置realdata到futuredata");
this.realData = my;
isRealData = true;
LockSupport.unpark(t);
} else {
return;
}
}
}
<file_sep>/src/cn/mumu/architecture/model/future/UserF.java
package cn.mumu.architecture.model.future;
public class UserF {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "UserF [name=" + name + "]";
}
}
<file_sep>/src/cn/mumu/architecture/model/MumuTest.java
package cn.mumu.architecture.model;
import cn.mumu.architecture.model.singleton.InnerClassSingleton;
public class MumuTest {
public static void main(String[] args) {
InnerClassSingleton instance = InnerClassSingleton.getInstance();
System.out.println(instance.hashCode());
}
}
| b08504ab8543e8edbb3fbe9ab3d59d1350c7c19e | [
"Java"
] | 3 | Java | hjl12/DDup | 9e0bc516b2c3db020c2cccf286362ff2d06432b3 | 49df819059e23aefd1cfe5b72fa5bff1c683a96a | |
refs/heads/master | <file_sep>package com.anuj.springboot.aop;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.EnableAspectJAutoProxy;
import com.anuj.springboot.aop.model.Strudent;
@SpringBootApplication
@EnableAspectJAutoProxy(proxyTargetClass=true)
public class SpringBootAopIntegrationApplication implements CommandLineRunner{
@Autowired
Strudent s;
public static void main(String[] args) {
SpringApplication.run(SpringBootAopIntegrationApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
System.out.println("Inside run");
System.out.println("To test git");
System.out.println("To test git clone");
s.setName("<NAME>");
s.getName();
s.setAge("25");
}
}
<file_sep>package com.anuj.springboot.aop.aspectj;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.annotation.Pointcut;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class AspectProgram {
@Before(value = "execution(* setName(..)) and args(name)")
public void beforeAdvice(JoinPoint joinPoint,String name) {
System.out.println(" before advice executed name:"+name+"joinPoint:"+joinPoint);
}
@After(value = "execution(* setName(..)) and args(name)")
public void afterAdvice(JoinPoint joinPoint,String name) {
System.out.println(" After advice executed name:"+name);
}
@Around(value = "execution(* setAge(..)) and args(age)")
public void arroundAdvice(ProceedingJoinPoint procedingJointPoint,String age) throws Throwable {
System.out.println("Arround advice getting exceited before method invocation age:"+age);
procedingJointPoint.proceed();
System.out.println("Arround advice getting exceuted after method invocaton");
}
@Before(value = "allGetter()")
public void berforeGetterAdvice() {
System.out.println("Inside getter advice");
}
@Pointcut(value = "execution(* setName(..))")
public void allGetter() {
}
}
| 25d75467f88a878dcf560fdac867386462a33d7a | [
"Java"
] | 2 | Java | anuj2841/SecondRepo | 7ece9eba4ce149d89d46ea55239447082a0562e3 | aac06ab5dfe5437444efa80168c63258fbf2711d | |
refs/heads/master | <file_sep>/*
Author: <NAME>
*/
#include <iostream>
#include <stdlib.h>
#include <vector>
#include <time.h>
#include <cmath>
#include <queue>
#include <cstdio> //EXIT_SUCESS
using namespace std;
#include "State.h"
#include "Game.h"
int main()
{
srand (time(NULL));
cout <<"Hello This program is to get the numbers arranged in a certain order\n"
//<< "Hello This Program is just uses four basic command u 'up', j 'down', h'left and k'right' \n"
<< " 1 2 3\n"
<< " 4 0 5\n"
<< " 6 7 8\n";
Game g;
g.startGame();
return 0;
}
<file_sep>#ifndef GAME_H
#define GAME_H
const State idealState('w');
class Game
{
public:
Game() {}
virtual ~Game() {}
void startGame();
protected:
private:
void possibleStates(State);
bool seqSearch(State);
void choiceForMove(State&,char);
void choiceForMove(State&);
State posFunc(int,State,char);
State cases(int,int,int,State);
priority_queue<State> posStates;//Move it to private section
vector<State> posStore;
};
void Game::startGame()
{
State alpha;
// posStates.push_back(alpha);
int contain=0;
char cho;
cout << "Do you want to enter the numbers yourself(y/n)\n"
<< "NOTE that by pressing n and enter the computer will randomly arrange the numbers\n";
cin >>cho;
if (cho=='y')
alpha.getArr();
cout << "Hello please input the way you wish to go"<< endl;
cout << endl;
posStore.push_back(alpha);
posStore[0].printBoard();
char inp;
int stateNUM=0,moreThanOne;
// int checc=0;
State hol;
for(int k=0; !(alpha==idealState); k++)
{
//cin >> inp ;
alpha.printBoard();
cout << "Whats Your Next Move ?\n"<<endl;
cout << "N.B the manhattan distance is " <<alpha.ManCalc()<<endl;
possibleStates(alpha);
cout << "Your next possible moves are as follows"<<endl;
State hol;
stateNUM=1;
moreThanOne=0;
while (!posStates.empty())
{
hol=posStates.top();
hol.printBoard();
cout << "The manhattan distance for this State is "<< hol.ManCalc()<<"\nState Number is "<<stateNUM;
cout << " The number of visited states are " << posStore.size() << endl;
if (!seqSearch(hol)&&!(moreThanOne>=1))
{
cout << "\nState Number "<< stateNUM<<" is chosen for being effiecent"<<endl;
alpha = hol;
moreThanOne++;
}
cout << "------------------\n";
posStates.pop();
stateNUM++;
}
if (moreThanOne==0)
alpha=hol;
posStore.push_back(alpha);
cout << "\n\n\n";
while (!posStates.empty())
{
posStates.pop();
}
}
while (!posStore.empty())
{
posStore.pop_back();
}
}
void Game::possibleStates(State a)
{
int ind1,ind2;
State hold;
State temp;
hold=a;
temp=a;
a.searchNum(ind1,ind2,0);
if (ind1>0 && ind2>0)
{
choiceForMove(hold,'u');
posStates.push(hold);
hold=temp;
choiceForMove(hold,'h');
posStates.push(hold);
hold=temp;
if (ind1==1&&ind2==1)
{
choiceForMove(hold,'j');
posStates.push(hold);
hold=temp;
choiceForMove(hold,'k');
posStates.push(hold);
hold=temp;
}
else if (ind1<2)
{
choiceForMove(hold,'j');
posStates.push(hold);
hold=temp;
}
else if (ind2<2)
{
choiceForMove(hold,'k');
posStates.push(hold);
hold=temp;
}
}
else if (ind1 < 2 && ind2 < 2)
{
choiceForMove(hold,'j');
posStates.push(hold);
hold=temp;
choiceForMove(hold,'k');
posStates.push(hold);
hold=temp;
if (ind1>0)
{
choiceForMove(hold,'u');
posStates.push(hold);
hold=temp;
}
else if (ind2>0)
{
choiceForMove(hold,'h');
posStates.push(hold);
hold=temp;
}
}
else if (ind1==2 && ind2==0)
{
choiceForMove(hold,'u');
posStates.push(hold);
hold=temp;
choiceForMove(hold,'k');
posStates.push(hold);
hold=temp;
}
else if (ind1==0 && ind2==2)
{
choiceForMove(hold,'j');
posStates.push(hold);
hold=temp;
choiceForMove(hold,'h');
posStates.push(hold);
hold=temp;
}
}
void Game::choiceForMove(State &a,char input)
{
int x=0,y=0;
a.searchNum(x,y,0);
if (x>0 && input =='u')
{
swap(a.stateArr[x-1][y],a.stateArr[x][y]);
}
else if (x<2 && input == 'j')
{
swap(a.stateArr[x+1][y],a.stateArr[x][y]);
}
else if (y>0 && input == 'h')
{
swap(a.stateArr[x][y-1],a.stateArr[x][y]);
}
else if(y<2 && input == 'k')
{
swap(a.stateArr[x][y+1],a.stateArr[x][y]);
}
else if (input=='c')
{
possibleStates(a);
a=posStates.top();
while (!posStates.empty())
{
posStates.pop();
}
}
else
cout << "Please input a correct move"<<endl;
}
void Game::choiceForMove(State &a)
{
int x=0,y=0;
a.searchNum(x,y,0);
if (x>0)
{
swap(a.stateArr[x-1][y],a.stateArr[x][y]);
}
else if (x<2)
{
swap(a.stateArr[x+1][y],a.stateArr[x][y]);
}
else if (y>0)
{
swap(a.stateArr[x][y-1],a.stateArr[x][y]);
}
else if(y<2)
{
swap(a.stateArr[x][y+1],a.stateArr[x][y]);
}
else
cout << "Please input a correct move"<<endl;
}
bool Game::seqSearch(State item)
{
for ( vector <State> :: iterator i = posStore.begin(); i != posStore.end(); ++i)
{
if (item==*i)
return true;
}
return false;
};
#endif // GAME_H
<file_sep># Assignment-1-Fin
DSA
This program is just a 3 x 3 number game where you don't play at all. It's only purpose it to randomly arrange numbers from 0-8 into that 3 x 3
format to then change systematically change the numbers step by step to the desired arrangement by manhattans distance.
<file_sep>#ifndef STATE_H
#define STATE_H
#include <iostream>
#include <vector>
#include <stdlib.h> /* srand, rand */
#include <time.h>
using namespace std;
const int listSize=9;
class State
{
public:
State()
{
vector <int> numbComb;//Consider to move it to some function
for (int i = 0 ; i < listSize ; i ++)
{
numbComb.push_back(i);
}
int randIndex,holder,begining;
int randCount=0;
for (int i = 0 ; i < listSize ; i ++)
{
randIndex=rand()%listSize;
randCount++;
// cout << "random index " << randIndex <<endl;
holder=numbComb[randIndex];
numbComb.erase(numbComb.begin()+randIndex);
numbComb.insert(numbComb.begin()+1,holder);
begining=numbComb[0];
numbComb.erase(numbComb.begin());
numbComb.insert(numbComb.begin()+randIndex,begining);
}
for (int i = 0 ; i < 3 ; i++)
{
for (int j = 0 ; j < 3 ; j++)
{
stateArr[i][j]=numbComb[3*i+j];
}
}
int h=1;
for (int i = 0 ; i < 3 ; i++)
{
for (int j = 0 ; j < 3 ; j++)
{
goal[i][j]=h;
h++;
}
}
goal[2][2]=0;
}
State(char s)
{
int z=1;
for (int i = 0 ; i < 3 ; i++)
{
for (int j = 0 ; j < 3 ; j++)
{
stateArr[i][j]=z;
z++;
}
}
stateArr[2][2]=0;
}
void printBoard()const;
bool operator==(const State&) const;
int ManCalc()const;
void getArr();
void searchNum(int&,int&,int)const;
int stateArr[3][3];
bool operator<(const State& s)const{
return s.ManCalc()<ManCalc();
}
virtual ~State() {}
protected:
private:
int goal[3][3];
bool seqSearchForNum(int a);
void searchNumGoal(int&,int&,int)const;
};
void State::getArr(){
int num=0;
for (int i = 0 ; i < 3 ; i ++)
for (int j = 0 ; j < 3 ; j++)
stateArr[i][j]=9;
for (int i = 0 ; i < 3 ; i ++)
for (int j = 0 ; j < 3 ;j++){
do {
cout << "Please input the array number, You are on number " << i*3+j<<endl;
cin >> num;
if (seqSearchForNum(num)){
system("CLS");
cout << "ERROR You inputed this number before please try again"<<endl;
}
}while(seqSearchForNum(num));
stateArr[i][j]=num;
}
}
bool State::operator==(const State& one)const
{
for (int i = 0 ; i < 3 ; i ++)
for (int j = 0 ; j < 3 ; j++)
if (stateArr[i][j]!=one.stateArr[i][j])
return false;
return true;
}
void State::printBoard()const
{
for (int i = 0 ; i < 3 ; i++)
{
for (int j = 0 ; j < 3 ; j ++)
cout << stateArr[i][j]<< " ";
cout << endl;
}
}
void State::searchNum(int &x,int &y,int num)const
{
for (int i = 0; i < 3 ; i ++)
for (int j = 0 ; j < 3; j++)
{
if (stateArr[i][j]==num){
x=i;
y=j;
}
}
}
void State::searchNumGoal(int &x,int &y,int num)const
{
for (int i = 0; i < 3 ; i ++)
for (int j = 0 ; j < 3; j++)
{
if (goal[i][j]==num){
x=i;
y=j;
}
}
}
int State::ManCalc()const{
int x2=0,y2=0,x1=0,y1=0;
int manDis=0;
for (int i = 0 ; i < 9 ; i++){
searchNumGoal(x2,y2,i);
searchNum(x1,y1,i);
manDis+=(abs((x1-x2))+abs((y1-y2)));
}
return manDis;
}
bool State::seqSearchForNum(int a)
{
for ( int i = 0; i < 3; i++)
{
for (int j = 0 ; j < 3 ; j++)
if (a==stateArr[i][j])
return true;
}
return false;
};
#endif // STATE_H
| 010d9ab904c65fe55ba6cd6000d8c8c333619d40 | [
"Markdown",
"C++"
] | 4 | C++ | mini96/Assignment-1-Fin | 0fa8e8c0e1995b5f1e336d374cb3b3de2eab4adb | bb734708fb06572fba5e00688fa719c57b2c89ef | |
refs/heads/master | <repo_name>Wil-Torres/ProyectoFinalSO1<file_sep>/src/principal/LeerArchivo.java
package principal;
import java.io.BufferedReader;
import java.io.FileReader;
public class LeerArchivo {
public LeerArchivo() {
// TODO Auto-generated constructor stub
}
public String[] lectura(String ruta){
BufferedReader lector;
String separador = ",";
String[] resultado = null;
try {
lector = new BufferedReader(new FileReader(ruta));
String lecturaLinea = lector.readLine();
while (lecturaLinea != null ) {
resultado = lecturaLinea.split(separador);
System.out.println(resultado);
lecturaLinea = lector.readLine();
}
lector.close();
return resultado;
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
<file_sep>/src/principal/Comensal.java
package principal;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Date;
import java.util.List;
import java.util.Random;
import java.util.concurrent.Semaphore;
public class Comensal extends Thread {
// declaramos las propiedades de la clase
private final int numero;
private final Semaphore[] palilloS;
private final List<List<Integer>> palilloComensal;
private final String nombre;
private final int izquierdo;
private final int derecho;
public Comensal(int numero, Semaphore[] palilloS, List<List<Integer>> palilloComensal, String nombre) {
this.numero = numero;
this.palilloS = palilloS;
this.palilloComensal = palilloComensal;
this.nombre = nombre;
this.izquierdo = palilloComensal.get(numero).get(0);
this.derecho = palilloComensal.get(numero).get(1);
}
@Override
public void run() {
while (Visualizar.corriendo) {
try {
comensalPensando();
comensalComiendo();
comensalDurmiendo();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
private void comensalDurmiendo() {
try {
// pensara durante un segundo
Comensal.sleep(Constantes.tiempoDurmiendo);
System.out.println("Filosofo " + nombre + " Durmiendo");
// cambiar emoji a durmiendo.
Visualizar.setEstado(numero, "dormir");
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
}
}
private void comensalComiendo() throws IOException {
//concedemos permiso para tomar el cubierto
if(palilloS[izquierdo].tryAcquire()){
if(palilloS[derecho].tryAcquire()){
System.out.println("Filosofo " + nombre + " inicia comiendo " + (new Date()));
enviartexto("Filosofo " + nombre + " inicia comiendo " + (new Date()));
Visualizar.setEstadoC(numero, "comer");
Visualizar.setMano(izquierdo, "mano");
Visualizar.setMano(derecho, "mano");
try {
sleep(new Random().nextInt(Constantes.tiempoMaximo) + Constantes.tiempoComiendo);
} catch (Exception e) {
// TODO: handle exception
}
// soltamos el cubierto para que lo utilice alguien mas
System.out.println("Filosofo " + nombre + " termina de comer " + (new Date()));
enviartexto("Filosofo " + nombre + " termina de comer " + (new Date()));
Visualizar.setEstado(numero, "sin_estado");
Visualizar.setMano(izquierdo, "vacio");
Visualizar.setMano(derecho, "vacio");
palilloS[derecho].release();
}
// soltamos el cubierto para que lo utilice alguien mas
palilloS[izquierdo].release();
} else {
System.out.println("Esperando turno");
Visualizar.setEstado(numero, "sin_estado");
}
}
private void comensalPensando() {
// TODO Auto-generated method stub
try {
// pensara durante un segundo
Comensal.sleep(Constantes.tiempoPensando);
System.out.println("Filosofo " + nombre + " pensando");
// cambiar emoji a pensando.
Visualizar.setEstado(numero, "pensar");
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
}
}
private void enviartexto(String texto) throws IOException{
String ruta = "/Users/wtorres/Desktop/archivo.txt";
File archivo = new File(ruta);
BufferedWriter bw;
if(archivo.exists()) {
bw = new BufferedWriter(new FileWriter(archivo));
bw.newLine();
bw.write(texto);
} else {
bw = new BufferedWriter(new FileWriter(archivo));
bw.write(texto);
}
bw.close();
}
}
<file_sep>/src/principal/Constantes.java
package principal;
public class Constantes {
public static int tiempoPensando = 1000;
public static int tiempoDurmiendo = 1000;
public static int tiempoComiendo = 1000;
public static int tiempoMaximo = 2000;
}
| d5f1d3e2ab017980545407dd0ee42682c3cdce34 | [
"Java"
] | 3 | Java | Wil-Torres/ProyectoFinalSO1 | 2fede24d4989f77acd7da4df1ecdee7eaa34f6f9 | b83529f6f5169bb5b217a6de494359ea3c68e248 | |
refs/heads/main | <repo_name>PhooMagoo/game_releases<file_sep>/game_releases.py
# Collect information about upcoming game releases.
from tkinter import Tk
import pyautogui
import re
import pandas as pd
from selenium import webdriver
from pprint import pprint
# Set up our variables.
newDates = []
newNames = []
copyText = ""
i = 0
# Get the site we're gonna take info from.
site_url = "https://gamefaqs.gamespot.com/new"
# How we're gonna find the games and releases.
regexName = re.compile('<div class="sr_name"><a href="(.*?)">(.*?)</a>')
regexDate = re.compile('<div class="sr_info">(.*)')
# Let's open the bad boy.
browser = webdriver.Chrome()
browser.get(site_url)
# Copy the HTML from our specified site.
pyautogui.rightClick(870, 480)
pyautogui.click(940, 720)
pyautogui.click(940, 720)
pyautogui.hotkey('ctrl', 'a')
pyautogui.hotkey('ctrl', 'c')
# Shove that HTML into an object.
res = Tk().clipboard_get()
# Filter out everything but the names and dates of new / upcoming releases.
names = regexName.findall(res)
dates = regexDate.findall(res)
# We have some straggler HTML, so we strip out just the name.
for x, y in names:
newNames.append(y)
# We have some straggler HTML for some of the dates, so we get rid of that.
for date in dates:
if "</div>" in date:
newDates.append(date.replace("</div>", ""))
else:
newDates.append(date)
# Format the text in a pleasing way, and...
for name in newNames:
copyText += newNames[i] + " : " + newDates[i] + ", \n"
i = i + 1
# ...shove it into our game_releases file.
with open("game_releases.txt", "w") as f:
f.write(copyText)
| 043686fe26e4031770cc4aa3ca84cfac07dd20d8 | [
"Python"
] | 1 | Python | PhooMagoo/game_releases | 5356c88bf322981cde27c850ae092a7113b0e47f | b69cf926db3d488600705bac145d0c88ef6723be | |
refs/heads/main | <repo_name>tanoshiibot/the-hangman<file_sep>/README.md
# The Hangman
Exercice BeCode : réalisation d'un pendu en js.
*<NAME>*
## Comment jouer ?
Vous pouvez jouer à la version HTML du jeu [ici](https://tanoshiibot.github.io/the-hangman/). Cependant, cette version ne servait que de débug avant de me lancer sur la version pour bot Discord. Pour le lancer, intégrez le code à votre bot (vous aurez besoin de discord.js).
**index.html**/**script.js**/**style.css** et le dossier **img** concernent la version HTML.
**main.js**/**hangman.js** concernent la version pour bot Discord.
### Jouer au pendu sur Discord :
* Pour démarrer le pendu, écrivez `>pendu start`. Le bot va générer un pendu. Utilisez également cette commande pour redémarrer une partie.

* Écrivez ensuite `>pendu [votre proposition de lettre]` pour proposer une lettre. Le dessin du pendu va se continuer si le mot ne contient pas la lettre, et placera la lettre dans les cases correspondantes si elle s'y trouve.
![Screenshot: >pendu [proposition de lettre]](https://github.com/tanoshiibot/the-hangman/blob/main/screenshots/pendu.PNG)
*(Si vous écrivez un nombre, une suite de lettre ou un caractère spécial, vous gâchez une vie. Les mots sont normalisés et ne contiennent pas d'accent ou d'espace.)*
* La partie se termine si le dessin du pendu se complète (perdu) ou si vous avez trouvé toutes les lettres (gagné). Vous devez refaire `>pendu start` pour relancer une partie.


## Difficultés rencontrées
J'ai pu utiliser ce projet pour apprendre les Regex. Il s'agit d'un outil très puissant, et je me rends compte qu'il résoud beaucoup de problèmes que j'ai déjà rencontré auparavant.
J'ai essayé de mettre la liste de mots sur mon serveur et de la récupérer depuis mon serveur, mais je n'ai pas réussi à temps...
## Améliorations possibles
* Le pendu est "prêt" à recevoir et trier une liste de mots bien plus complète que la lsite actuelle. Cependant, il faut que j'apprenne à accder à cette liste de mot autrement qu'en local ou sur la RAM, car cette liste est gigantesque.
* J'aurais également aimé qu'on puisse choisir l'émoji pendu, mais ce n'était pas ma priorité. Je le rajouterai peut-être car c'est assez simple à rajouter.
* Je devrais afficher le mot lorsque la partie est perdue.
<file_sep>/script.js
let words = ["moka", "tiramisu", "panna cotta", "caramel", "capucinno", "crêpe", "latte machiatto", "mille-feuille", "merveilleux", "cupcake", "chocolat", "cheesecake", "meringue", "gâteau", "cookie", "madelaine", "sablé", "palmier", "brownie", "vanille", "crème", "spéculoos", "flan", "pudding", "donut", "beignet", "sucre", "café", "stretto", "lungo", "doppio", "expresso", "crème brûlée", "moelleux", "cannolis", "matcha", "sencha", "genmaicha", "gyokuro"]
words = words.filter(element => ((!/[ 0-9]/.test(element)) && (element.length > 4)));
let word = [];
let spaces = [];
let keysPressed = [];
let lives = 9;
let missChara = 0;
function accentsTidy(s) {
let r = s.toLowerCase();
r = r.replace(new RegExp(/\s/g),"");
r = r.replace(new RegExp(/[àáâãäå]/g),"a");
r = r.replace(new RegExp(/æ/g),"ae");
r = r.replace(new RegExp(/ç/g),"c");
r = r.replace(new RegExp(/[èéêë]/g),"e");
r = r.replace(new RegExp(/[ìíîï]/g),"i");
r = r.replace(new RegExp(/ñ/g),"n");
r = r.replace(new RegExp(/[òóôõö]/g),"o");
r = r.replace(new RegExp(/œ/g),"oe");
r = r.replace(new RegExp(/[ùúûü]/g),"u");
r = r.replace(new RegExp(/[ýÿ]/g),"y");
r = r.replace(new RegExp(/\W/g),"");
return r;
};
lives = 9;
word = [...words[Math.floor(words.length * Math.random())]];
word.forEach((x, i) => {
word[i] = accentsTidy(x);
})
spaces = [...word].map(x => x == "-" ? " - " : " _ ");
missChara = word.length;
spaces.forEach((x) => {
let a = document.createElement("LI");
a.innerText = x;
a.setAttribute("class", "character");
document.getElementById("word").appendChild(a);
if (x == " - ") {
missChara--;
}
})
document.getElementById("debug").innerText = `${lives} left before death !`;
document.addEventListener("keydown", event => {
if ((lives > 0) && (missChara > 0)) {
if ((event.keyCode >= 65) && (event.keyCode <= 90)) {
document.getElementById("output").innerText = event.key;
if (keysPressed.includes(event.key)){
document.getElementById("output2").innerText = "déjà dedans !!!";
} else {
keysPressed.push(event.key);
if (word.includes(event.key)) {
word.forEach((x, i) => {
if (x == event.key) {
document.getElementsByClassName("character")[i].innerText = ` ${event.key} `;
missChara--;
document.getElementById("output2").innerText = missChara;
if (missChara == 0) {
document.getElementById("output").innerText = "your won :)";
}
}
})
} else {
lives--;
document.getElementById("hangman").setAttribute("src", `./img/${-lives + 9}.png`);
}
}
}
document.getElementById("debug").innerText = `${lives} left before death !`
} else if (lives == 0) {
document.getElementById("output").innerText = "your ded :(";
}
})
<file_sep>/hangman.js
module.exports = function generateHangman() {
let words = ["moka", "tiramisu", "panna cotta", "caramel", "capucinno", "crêpe", "latte machiatto", "mille-feuille", "merveilleux", "cupcake", "chocolat", "cheesecake", "meringue", "gâteau", "cookie", "madelaine", "sablé", "palmier", "brownie", "vanille", "crème", "spéculoos", "flan", "pudding", "donut", "beignet", "sucre", "café", "stretto", "lungo", "doppio", "expresso", "crème brûlée", "moelleux", "cannolis", "matcha", "sencha", "genmaicha", "gyokuro"]
words = words.filter(element => ((!/[ 0-9]/.test(element)) && (element.length > 4)));
let word = [];
let spaces = [];
let lives = 9;
let missChara = 0;
function accentsTidy(s) {
let r = s.toLowerCase();
r = r.replace(new RegExp(/[àáâãäå]/g),"a");
r = r.replace(new RegExp(/æ/g),"ae");
r = r.replace(new RegExp(/ç/g),"c");
r = r.replace(new RegExp(/[èéêë]/g),"e");
r = r.replace(new RegExp(/[ìíîï]/g),"i");
r = r.replace(new RegExp(/ñ/g),"n");
r = r.replace(new RegExp(/[òóôõö]/g),"o");
r = r.replace(new RegExp(/œ/g),"oe");
r = r.replace(new RegExp(/[ùúûü]/g),"u");
r = r.replace(new RegExp(/[ýÿ]/g),"y");
return r;
};
lives = 9;
word = [...words[Math.floor(words.length * Math.random())]];
word.forEach((x, i) => {
word[i] = accentsTidy(x);
})
spaces = [...word].map(x => x == "-" ? " - " : " ? ");
missChara = word.length;
spaces.forEach((x) => {
if (x == " - ") {
missChara--;
}
})
let hangman = [word, spaces, missChara];
return hangman;
} | e47ec946bbaa4e5b7f3febf27305d0ceeb59ee15 | [
"Markdown",
"JavaScript"
] | 3 | Markdown | tanoshiibot/the-hangman | f0648c6015e868fd976103daed47a65487e17f1b | 73c003d1db68f810a7fb84469219996b1c86bbb2 | |
refs/heads/master | <file_sep># What Every Developer Should Know About SQL Server Performance
This github repository is a complement to my Pluralsight course [http://bit.ly/SqlPerformanceCourse] ("What Every Developer Should Know Aboust SQL Server Performance"). In addition to my Pluralsight course, I also do a conference talk by the same name that shares much of the same content, so this repository supports both the course and the talk
## Requirements
You need to have a SQL Server version of 2008R@ or later available. SQL Express on your local workstation will work fine. If you don't have SQL Server express, you can download SQL Server Express 2016 from the site [downloadsqlserverexpress.com](http://www.hanselman.com/blog/DownloadSqlServerExpress.aspx). This is a blog post from <NAME>, but contains links directly to the correct download pages which is much easier than searching around Microsoft's web site.
## Sample Database
The sample database used throughout the course and talk is based on a fictional university to track what students have applied, enrolled and their progress in their courses. The database has enough data so you will get some "real" execution plans and can try out troubleshooting some real performance problems for yourself.
The sample database is provided in two ways:
1) As MS SQL backup files
2) as bcp raw data that you can import using the bcp utility.
Using a backup file is probably the easiest, but bcp data is a surefire way to get the data if for some reason you run into trouble restoring backups
### Restoring a backup file to create the sample database
There are separate backup files for SQL Server 2008R2, SQL Server 2012 and SQL Server 2014. If you are on SQL Server 2016, you should be able to use the SQL 2014 file.
Once you have the appropriate file downloaded, unzip the file. Then, in SQL Server Management Studio, right click on the databases folder and select "Restore Database". In the dialog box, choose "Device" and locate the file ("Students.bak") file you just unzipped. Follow the prompts and the database will be restored to your system.
### Using the bcp data to create the sample database
If you have trouble with the backup file, or just prefer to import your data directly, I've put together a zip file of the raw data exported out using the SQL Server bcp utility. In this file, I have a readme.txt file that tells the exact steps to bring the data in, but basically, you will do the following:
1) Go into Management Studio and create a new database called Students.
2) R un the included Students-Schema.sql file in Management Studio to create all of the schema objects (tables)
3) At a command prompt, run a series of bcp commands to bring the data into SQL Server. In the file, there is a list of all the commands you need to run in the correct order.
## Dynamic Management View Queries
The Dynamic Management Views contain a wealth of useful information for performance troubleshooting. While many DMV's are targetted at DBA's, they can also be very useful for developers to look at, so I have included some of the most useful queries here. These are all in the folder labeled DMV queries.
Note: You must have VIEW SERVER STATE permission to access these views. This is something you may need to get your DBA to grant to you. If they are unwilling to do this in a production environment, you can always as them to run one of these queries for you and send you the results. What is important is you know such information exists so you can ask for it when needed.
If you are looking for some description of these queries, I have a number of blog posts on these which you can access at [http://buildingbettersoftware.blogspot.com/2016/04/dmv-queries-from-my-pluralsight-course.html] (http://buildingbettersoftware.blogspot.com/2016/04/dmv-queries-from-my-pluralsight-course.html)
<file_sep>SELECT
login_name,
host_name,
host_process_id,
COUNT(1) As LoginCount
FROM sys.dm_exec_sessions
WHERE is_user_process = 1
GROUP BY
login_name,
host_name,
host_process_id; | 7bc4d0d808e2880c9bd8d23ea0577effac3731de | [
"Markdown",
"SQL"
] | 2 | Markdown | slash2382/SqlServerPerformance | 305da33b14d03e4b0e65f7d849efcfbc89b9b5ff | 13af15cb3c3ca9f1737d212f6b9227cc230dae9e | |
refs/heads/master | <file_sep>const app = require('../app');
const request = require('supertest');
describe('gae_node_request_example', () => {
describe('GET /helloworld', () => {
it('should get 200', done => {
request('http://127.0.0.1:8080')
.get('/helloworld')
.expect(200, done);
});
it('should get Hello World', done => {
request('http://127.0.0.1:8080')
.get('/helloworld')
.expect('{"data":{"hello":"Hello world!"}}', done);
});
});
});
<file_sep>'use strict';
Package('org.quickcorp.custom.backend.helloworld',[
Class('Microservice',BackendMicroservice,{
get:function (data){
let microservice = this;
var { graphql, buildSchema } = require('graphql');
// Construct a schema, using GraphQL schema language
var schema = buildSchema(`
type Query {
hello: String
}
`);
// The root provides a resolver function for each API endpoint
var root = {
hello: () => {
return 'Hello world!';
},
};
// Run the GraphQL query '{ hello }' and print out the response
graphql(schema, '{ hello }', root).then((response) => {
microservice.body = response;
microservice.done();
});
}
})
]);
<file_sep># Making a GraphQL Micro-service with QCObjects HTTP2 Built-In Server
In the following step-by-step tutorial I'll show you how to make a fancy, clean and quick micro-service using QCObjects HTTP2 Built-In Server and GraphQL
If you want to know more about GraphQL go to [https://graphql.org](https://graphql.org)
If you want to learn more about QCObjects go to [https://qcobjects.dev](https://qcobjects.dev)
1.- Create a progressive web app using the command line of QCObjects CLI Tool (you should be inside the project's folder):
```shell
> qcobjects create --pwa mynewmicroservice
```
2.- Install GraphQL inside the project's folder:
```shell
> npm install graphql --save
```
3.- Create/Edit a config.json file inside the project's folder
```shell
> vi config.json
```
4.- In the new config.json file add the following lines:
```json
{
"relativeImportPath": "js/packages/",
"domain": "0.0.0.0",
"backend": {
"routes": [{
"path": "/helloworld",
"microservice": "org.quickcorp.custom.backend.helloworld"
}]
}
}
```
5.- Then create/edit a new package inside the js/packages folder:
```shell
> vi js/packages/org.quickcorp.custom.backend.helloworld.js
```
6.- The following animation shows the basic code of a Microservice using GraphQL and QCObjects HTTP2 Built-In Server
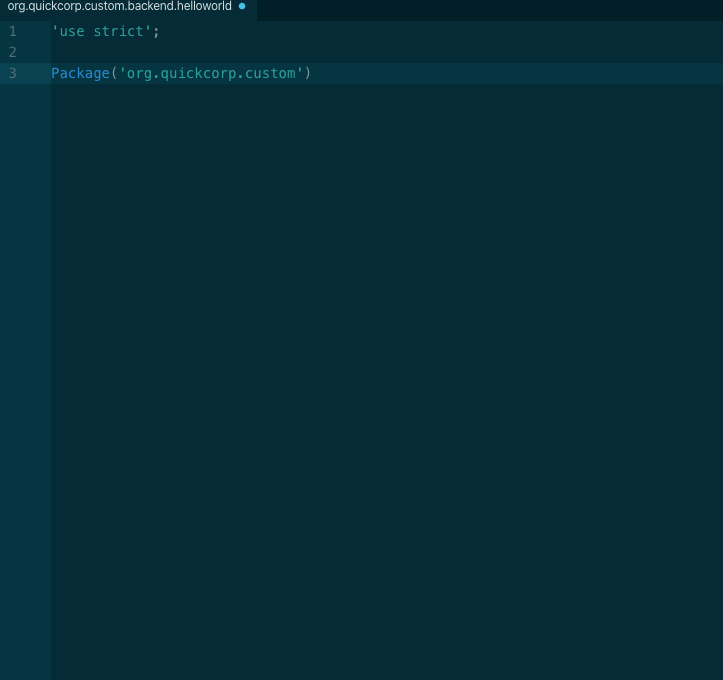
7.- Launch the QCObjects HTTP2 Built-In Server using the following command line:
```shell
> qcobjects launch mynewmicroservice
```
8.- Then open a browser in the address https://127.0.0.1/helloworld and you should see a result like this:
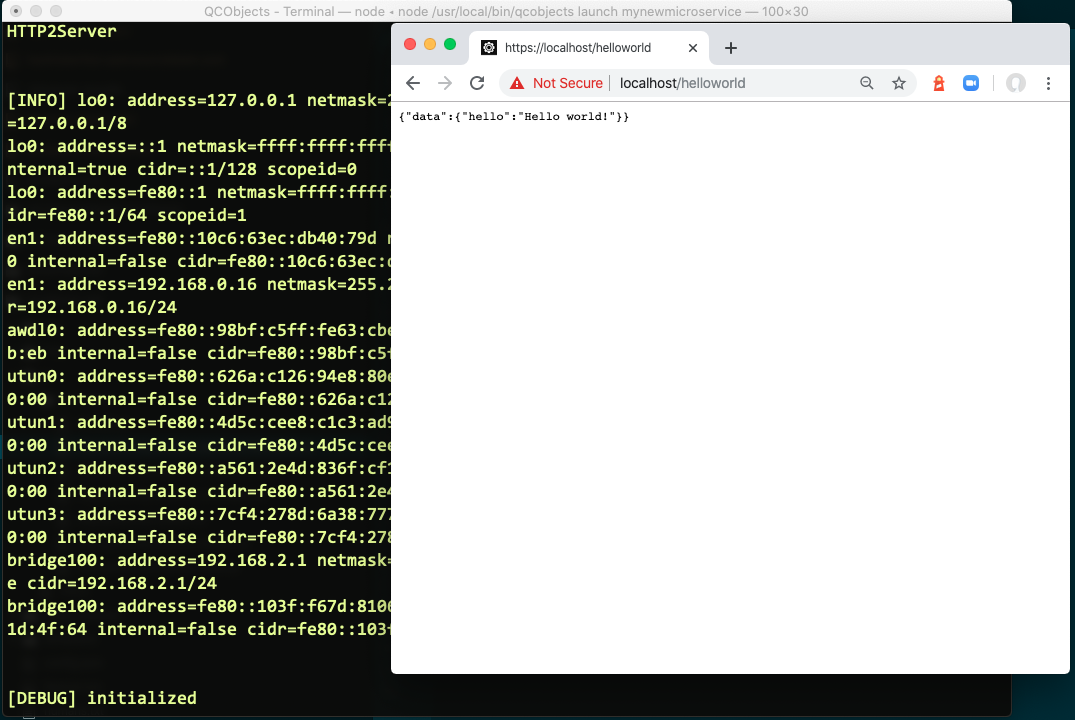
| 26c55a21c609786583bda8eb9d05f8bad077c46e | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | jeanmachuca/qcobjects-graphql-example | 023e0aadf6abfb3d0c81a5e6c2e384eb001dd56e | 405e6ab7cbdfd8e925e2c7cd48f55773e33c4217 | |
refs/heads/master | <file_sep>
// public class Bird extends Animal {
// private String kind = "鳥";
// public String getKind(String kind){
// return kind;
// }
// }
class Bird extends Animal{
public String getKind(){
return "鳥";
}
}
<file_sep>class While_Loop {
public static void main(String[] args){
int total = 0;
// カウント変数の初期化
int i = 1;
// 1〜100まで加算する。
while(i<=100){
total += i;
i++; //カウント変数のインクリメント
}
System.out.println("1〜100までの合計は"+total+"です。");
}
}
<file_sep>class Mary extends Enemy{
public Mary(){
this.winPoint = 3;
this.losePoint = 9;
this.name = "メアリー";
}
public boolean Battle(){
boolean result = false;
int loseNum = this.r.nextInt(5);
System.out.print("メアリー「1〜5のうち、外れは1つわよ」(1〜5を入力):");
while(true){
String line = this.sc.nextLine();
int userNum = 0;
//���l�`�F�b�N
try {
userNum = Integer.parseInt(line);
if(userNum >= 1 && userNum <= 5){
System.out.println("外れは" + String.valueOf(loseNum + 1));
if(loseNum + 1 != userNum){
result = true;
}
break;
}
} catch (Exception ex) {
}
System.out.print("1〜5の数値を入力してください。:");
}
return result;
}
}
<file_sep>import java.util.Scanner;
import java.util.ArrayList;
public class Main{
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
// 動物のArrayListを作ってる?
ArrayList<Animal> list = new ArrayList<Animal>();
// ループの数は動物リストが3以外の時=3まで
while(list.size() != 3){
Animal ani = null;
System.out.print("種類を指定してください(1:猫 2:鳥):");
String kind = sc.nextLine();
if(kind.equals("1")){
// 型変換(キャスト)を行っている。
// Cat型→Animal型
// 型変換ができるということはAnimalは親クラスなんだということが分かる
ani = (Animal)new Cat();
}else if(kind.equals("2")){
ani = (Animal)new Bird();
}else{
// 種類のエラー
System.out.println("入力エラーです。");
System.out.println("プログラムを終了します。");
System.exit(0);
}
System.out.print("名前を入力してください:");
String name = sc.nextLine();
if(name.isEmpty()){
// 名前のエラー
System.out.println("入力エラーです。");
System.out.println("プログラムを終了します。");
System.exit(0);
}
ani.setName(name);
list.add(ani);
} // 入力ループの終わり
for(Animal ani :list){
System.out.println(ani.getName() + "は" + ani.getKind() + "です。");
}
System.out.println("プログラムを終了します。");
}
}
<file_sep>import java.io.*;
class If_ElseIf_Else {
public static void main(String[] args) throws IOException{
System.out.println("お買い上げ金額を入力してください。");
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String str = br.readLine();
int price = Integer.parseInt(str);
if(price>=3000){
System.out.println(price+"円のお買い上げです。");
System.out.println("駐車料金は0円です。");
}else if(price>=2000){
System.out.println(price+"円のお買い上げです。");
System.out.println("駐車料金は100円です。");
}else if(price>=1000){
System.out.println(price+"円のお買い上げです。");
System.out.println("駐車料金は200円です。");
}else{
System.out.println(price+"円のお買い上げです。");
System.out.println("駐車料金は300円です。");
}
}
}
<file_sep>class SalesTax{
public static void main(String[] args){
final double CONSUMPTION_TAX = 0.08;
int price = 10000;
int taxIncluded = (int) (price + (price * CONSUMPTION_TAX) );
System.out.println("税抜き価格: " + price);
System.out.println("税込み価格: " + taxIncluded);
}
}<file_sep>class For_In {
public static void main(String[] args){
if(args.length<2){
System.out.println("数値を2つ以上入力して下さい。");
System.exit(-1);
}
int total = 0;
int number = 0;
for(String str : args){
number = Integer.parseInt(str);
total += number;
}
System.out.println("******************************");
System.out.println("入力した数値の合計は : "+total);
System.out.println("******************************");
total = 0;
for(int i=0; (i<args.length)&&(i<5); i++){
number = Integer.parseInt(args[i]);
total += number;
}
System.out.println("*************************************");
System.out.println("入力した数値(最大5個)の合計は : "+total);
System.out.println("*************************************");
}
}
<file_sep>import java.util.Random;
class Main{
public static void main(String args[]){
// Playerのコンストラクトをよびプレイヤーを生成する
// ループ
// 敵をランダムで出現させる
// ループ終了
Player p = new Player(); //プレイヤー生成
Random r = new Random(System.currentTimeMillis()); //実行するたびにms単位でランダム生成している
while(true){
p.ShowStatus(); // ステータス
Enemy e = null;
// nextInt(3)とすると
switch(r.nextInt(3)){
case 0:
e = new Bob();
//e = (Enemy)new Bob(); Enemy型にキャストしている。
break;
case 1:
e = new Mary();
//e = (Enemy)new Mary(); Enemy型にキャストしている。
break;
case 2:
e = new Yamada();
//e = (Enemy)new Mary(); Enemy型にキャストしている。
break;
}
System.out.println(e.getName() + "が現れた!!");
if(e.Battle()){
// 勝利時
System.out.println(e.getName() + "に勝利!!" + String.valueOf(e.getWinPoint()) + "ポイント獲得!");
p.Win(e);
}else{
// 敗北時
System.out.println(e.getName() + "に敗北!!" + String.valueOf(e.getLosePoint()) + "ポイント取られた!");
p.Lose(e);
}
// 終了判定
if(p.getPoint() <= 0){
break;
}
}
p.ShowStatus();
System.out.println("ゲームオーバー");
}
}
<file_sep>
// public class Cat extends Animal{
// private String kind = "猫";
// public String getKind(String kind){
// return kind;
// }
// }
class Cat extends Animal{
public String getKind(){
return "猫";
}
// セッターはいらないの?
// 猫に対して犬と上書きできてはいけないので読み取り専用のゲッターだけになっている。
}
<file_sep>public class Answer1 {
public static void main(String[] args){
// エラーチェック
if(args.length != 3){
System.out.println("引数は3つ指定してください。");
return;
}
// 実行
System.out.println(args[2]);
System.out.println(args[1]);
System.out.println(args[0]);
// 以下でもOK
// for(int i=2; i>=0; i--){
// System.out.println(args[i]);
// }
}
}
<file_sep>class Variable {
public static void main(String[] args){
int diameter=10;
int radius=5;
double area;
System.out.println("直径は"+diameter);
System.out.println("半径は"+radius);
System.out.println("円の面積は"+radius*radius*3.14);
}
}
<file_sep>public class Prac {
public static void main(String[] args) {
if (args.length != 1) {
System.out.println("点数は1つだけ入力してください。");
System.exit(-1);
}
String rank = null;
int score = Integer.parseInt(args[0]);
if (score < 0 || score > 100) {
System.out.println("0〜100を入力してください。");
System.exit(-1);
}
if (score >= 90) {
rank = "A";
} else if (score >= 70) {
rank = "B";
} else if (score >= 50) {
rank = "C";
} else if (score >= 30) {
rank = "D";
} else {
rank = "E";
}
System.out.println("成績の結果は" + rank + "ランクです。");
}
}
<file_sep>class Question1{
public static void main(String[] args){
if (args.length > 3 || args.length < 3 ) {
System.out.println("引数は3つ指定してください。");
System.exit(-1);
}
int[] num = new int[3];
num[0] = Integer.parseInt(args[0]);
num[1] = Integer.parseInt(args[1]);
num[2] = Integer.parseInt(args[2]);
for(int i=2; i>=0; i--){
System.out.println(num[i]+"");
}
}
}<file_sep>import java.util.*;
// 抽象クラスなのでEnemyをnewできない。
abstract class Enemy{
public Enemy(){
r = new Random(System.currentTimeMillis());
sc = new Scanner(System.in);
}
protected Scanner sc;
protected Random r;
protected String name;
protected int winPoint;
protected int losePoint;
abstract boolean Battle();
public int getWinPoint(){
return this.winPoint;
}
public int getLosePoint(){
return this.losePoint;
}
public String getName(){
return this.name;
}
}
<file_sep>class Double_Loop {
public static void main(String[] args){
if(args.length!=2){
System.out.println("高さと幅を入力して下さい");
System.exit(-1);
}
int height = Integer.parseInt(args[0]);
int width = Integer.parseInt(args[1]);
System.out.println("高さは"+height+"、幅は"+width);
for(int i=0; i<height; i++){
for(int j=0; j<width; j++){
System.out.print("◯");
}
System.out.println();
}
}
}
<file_sep>class Intro{
public static void main(String[] args){
System.out.println("私は内藤誠です。");
System.out.println("好きなスポーツはゴルフです。");
System.out.println("好きな食べ物はタコスです。");
System.out.println("好きなアニメはラブライブです。");
System.out.println("一人前のSEになれるように修行中です。");
}
} | f17555ca9c6c4cd0ec043db724731334a5c38ec0 | [
"Java"
] | 16 | Java | natsuki525/java_work | 1b36159df403acfb882eb09caf00735f743ecd6b | ada02e67d03af3b670a7cdbaad4d9c1d16d55fcc | |
refs/heads/dev | <file_sep>using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using App1.Models;
namespace App1.Services
{
class MockBeerDataStore : IDataStore<Beer>
{
List<Beer> beers;
List<Beer> myBeers;
public MockBeerDataStore()
{
myBeers = new List<Beer>();
beers = new List<Beer>();
var mockBeers = new List<Beer>
{
new Beer
{
Name = "Tyskie",
Description =
"Gronie to wzorcowy przykład jasnego, pełnego piwa, które od lat cieszy się uznaniem nie tylko w Polsce, ale i na całym świecie."
}
};
foreach (var beer in mockBeers)
{
beers.Add(beer);
}
}
public async Task<bool> AddItemAsync(Beer item)
{
beers.Add(item);
return await Task.FromResult(true);
}
public async Task<bool> UpdateItemAsync(Beer item)
{
var oldItem = beers.Where((Beer arg) => arg.Id == item.Id).FirstOrDefault();
beers.Remove(oldItem);
beers.Add(item);
return await Task.FromResult(true);
}
public async Task<bool> DeleteItemAsync(int id)
{
var oldItem = beers.Where((Beer arg) => arg.Id == id).FirstOrDefault();
beers.Remove(oldItem);
return await Task.FromResult(true);
}
public async Task<Beer> GetItemAsync(int id)
{
return await Task.FromResult(beers.FirstOrDefault(s => s.Id == id));
}
public async Task<IEnumerable<Beer>> GetItemsAsync(bool forceRefresh = false)
{
return await Task.FromResult(beers);
}
public async Task<IEnumerable<Beer>> GetMyBeersAsync(bool forceRefresh = false)
{
return await Task.FromResult(myBeers);
}
public async Task<bool> AddMyBeer(Beer beer)
{
myBeers.Add(beer);
return await Task.FromResult(true);
}
}
}<file_sep>using System.IO;
using Android.App;
using Android.Content.PM;
using Android.OS;
using Xamarin.Forms;
using Xamarin.Forms.Platform.Android;
using Environment = System.Environment;
namespace App1.Droid
{
[Activity(Label = "App1", Icon = "@mipmap/icon", Theme = "@style/MainTheme", MainLauncher = true,
ConfigurationChanges = ConfigChanges.ScreenSize | ConfigChanges.Orientation)]
public class MainActivity : FormsAppCompatActivity
{
protected override void OnCreate(Bundle savedInstanceState)
{
TabLayoutResource = Resource.Layout.Tabbar;
ToolbarResource = Resource.Layout.Toolbar;
string dbName = "app_db.sqlite";
string folderPath = Environment.GetFolderPath(Environment.SpecialFolder.Personal);
string fullpath = Path.Combine(folderPath, dbName);
base.OnCreate(savedInstanceState);
Forms.Init(this, savedInstanceState);
LoadApplication(new App(fullpath));
}
}
}<file_sep>using System;
using App1.Models;
using Xamarin.Forms;
using Xamarin.Forms.Xaml;
namespace App1.Views
{
[XamlCompilation(XamlCompilationOptions.Compile)]
public partial class NewBeerPage : ContentPage
{
public Beer Beer { get; set; }
public NewBeerPage()
{
InitializeComponent();
Beer = new Beer
{
Name = "<NAME>",
Description = "This is a beer description."
};
BindingContext = this;
}
async void Save_Clicked(object sender, EventArgs e)
{
MessagingCenter.Send(this, "AddItem", Beer);
await Navigation.PopModalAsync();
}
}
}<file_sep>using System;
using App1.Models;
using App1.ViewModels;
using Xamarin.Forms;
using Xamarin.Forms.Xaml;
namespace App1.Views
{
[XamlCompilation(XamlCompilationOptions.Compile)]
public partial class BeerDetailPage : ContentPage
{
BeerDetailViewModel viewModel;
public Beer Beer { get; set; }
public BeerDetailPage(BeerDetailViewModel viewModel)
{
InitializeComponent();
BindingContext = this.viewModel = viewModel;
}
public BeerDetailPage()
{
InitializeComponent();
var Beer = new Beer
{
Name = "<NAME>",
Description = "This is an item description."
};
viewModel = new BeerDetailViewModel(Beer);
BindingContext = viewModel;
}
async void Add_Clicked(object sender, EventArgs e)
{
MessagingCenter.Send(this, "AddToMyBeer", viewModel.Beer);
}
}
}<file_sep>using SQLite;
namespace App1.Models
{
public class Beer
{
[PrimaryKey, AutoIncrement] public int Id { get; set; }
public string Name { get; set; }
public string Text { get; set; }
public string Description { get; set; }
public string Style { get; set; }
public Brewery Brewery { get; set; }
public int Rate { get; set; }
}
}<file_sep>using System;
using App1.Models;
using App1.ViewModels;
using Xamarin.Forms;
using Xamarin.Forms.Xaml;
namespace App1.Views
{
[XamlCompilation(XamlCompilationOptions.Compile)]
public partial class MyBeersPage : ContentPage
{
MyBeerViewModel viewModel;
public MyBeersPage()
{
InitializeComponent();
BindingContext = viewModel = new MyBeerViewModel();
}
async void OnBeerSelected(object sender, SelectedItemChangedEventArgs args)
{
var item = args.SelectedItem as Beer;
if (item == null)
return;
await Navigation.PushAsync(new MyBeerDetailPage(new BeerDetailViewModel(item)));
// Manually deselect item.
BeersListView.SelectedItem = null;
}
async void AddBeer_Clicked(object sender, EventArgs e)
{
await Navigation.PushModalAsync(new NavigationPage(new NewBeerPage()));
}
protected override void OnAppearing()
{
base.OnAppearing();
if (viewModel.MyBeers.Count == 0)
viewModel.LoadItemsCommand.Execute(null);
}
}
}<file_sep>using System;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Threading.Tasks;
using App1.Models;
using App1.Views;
using Xamarin.Forms;
namespace App1.ViewModels
{
public class MyBeerViewModel : BaseViewModel
{
public Command LoadItemsCommand { get; set; }
public ObservableCollection<Beer> MyBeers { get; set; }
public MyBeerViewModel()
{
Title = "MyBeers";
MyBeers = new ObservableCollection<Beer>();
LoadItemsCommand = new Command(async () => await ExecuteLoadBeersCommand());
MessagingCenter.Subscribe(this, "AddToMyBeer",
(Action<BeerDetailPage, Beer>) (async (obj, item) =>
{
var newItem = item;
MyBeers.Add(newItem);
await BeerStore.AddMyBeer(newItem);
}));
}
async Task ExecuteLoadBeersCommand()
{
if (IsBusy)
{
return;
}
IsBusy = true;
try
{
MyBeers.Clear();
var beers = await BeerStore.GetMyBeersAsync(true);
foreach (var beer in beers)
{
MyBeers.Add(beer);
}
}
catch (Exception ex)
{
Debug.WriteLine(ex);
}
finally
{
IsBusy = false;
}
}
}
}<file_sep>using System;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Threading.Tasks;
using App1.Models;
using App1.Views;
using Xamarin.Forms;
namespace App1.ViewModels
{
public class BeersViewModel : BaseViewModel
{
public ObservableCollection<Beer> Beers { get; set; }
public Command LoadBeersCommand { get; set; }
public BeersViewModel()
{
Title = "Browse";
Beers = new ObservableCollection<Beer>();
LoadBeersCommand = new Command(async () => await ExecuteLoadItemsCommand());
MessagingCenter.Subscribe(this, "AddItem",
(Action<NewBeerPage, Beer>) (async (obj, item) =>
{
var newItem = item;
Beers.Add(newItem);
await BeerStore.AddItemAsync(newItem);
}));
}
async Task ExecuteLoadItemsCommand()
{
if (IsBusy)
return;
IsBusy = true;
try
{
Beers.Clear();
var items = await BeerStore.GetItemsAsync(true);
foreach (var item in items)
{
Beers.Add(item);
}
}
catch (Exception ex)
{
Debug.WriteLine(ex);
}
finally
{
IsBusy = false;
}
}
}
}<file_sep>using System.Collections.Generic;
using SQLite;
namespace App1.Models
{
public class Brewery
{
[PrimaryKey, AutoIncrement] public int Id { get; set; }
public string Text { get; set; }
public string Description { get; set; }
public List<Beer> BeerList { get; set; }
}
}<file_sep>using SQLite;
namespace App1.Models
{
public class Item
{
[PrimaryKey, AutoIncrement] public int Id { get; set; }
public string Text { get; set; }
public string Description { get; set; }
}
}<file_sep>using App1.Models;
namespace App1.ViewModels
{
public class BeerDetailViewModel : BaseViewModel
{
public Beer Beer { get; set; }
public BeerDetailViewModel(Beer item = null)
{
Title = item?.Name;
Beer = item;
}
}
}<file_sep>using App1.ViewModels;
using Xamarin.Forms;
using Xamarin.Forms.Xaml;
namespace App1.Views
{
[XamlCompilation(XamlCompilationOptions.Compile)]
public partial class MyBeerDetailPage : ContentPage
{
BeerDetailViewModel viewModel;
public MyBeerDetailPage(BeerDetailViewModel viewModel)
{
InitializeComponent();
BindingContext = this.viewModel = viewModel;
}
// public MyBeerDetailPage()
// {
// InitializeComponent();
//
// var Beer = new Beer
// {
// Name = "<NAME>",
// Description = "This is an item description."
// };
//
// viewModel = new BeerDetailViewModel(Beer);
// BindingContext = viewModel;
// }
// async void Update(object sender, EventArgs e)
// {
// MessagingCenter.Send(this, "AddToMyBeer", viewModel.Beer);
// }
}
}<file_sep>using System;
using App1.Models;
using App1.ViewModels;
using Xamarin.Forms;
using Xamarin.Forms.Xaml;
namespace App1.Views
{
[XamlCompilation(XamlCompilationOptions.Compile)]
public partial class BeersPage : ContentPage
{
BeersViewModel viewModel;
public BeersPage()
{
InitializeComponent();
BindingContext = viewModel = new BeersViewModel();
}
async void OnItemSelected(object sender, SelectedItemChangedEventArgs args)
{
var beers = args.SelectedItem as Beer;
if (beers == null)
return;
await Navigation.PushAsync(new BeerDetailPage(new BeerDetailViewModel(beers)));
// Manually deselect item.
BeersListView.SelectedItem = null;
}
async void AddItem_Clicked(object sender, EventArgs e)
{
await Navigation.PushModalAsync(new NavigationPage(new NewBeerPage()));
}
protected override void OnAppearing()
{
base.OnAppearing();
if (viewModel.Beers.Count == 0)
viewModel.LoadBeersCommand.Execute(null);
}
}
} | 01ee6b8a4ae8308887783fca0f58e3dfce63b265 | [
"C#"
] | 13 | C# | Meliousas/brewery-search-app | 73d774d061c5afd675f5af97a6197a55eb4da260 | 60645b120bcd0fd3cce50ef8b97133f31611d9e2 | |
refs/heads/master | <repo_name>LabNeuroCogDevel/BarsBTC<file_sep>/00_getBars.bash
rsync -Lrzvhi foranw@wallace:/data/Luna1/Raw/MRCTR/ subjs/ \
--include='[0-9][0-9][0-9][0-9][0-9]_[0-9][0-9][0-9][0-9][0-9][0-9][0-9][0-9]' \
--include='*/*mprage*' \
--include='*/*BarsReward*/' \
--include='*/*mprage*/*' \
--include='*/*BarsReward*/*' \
--exclude='*' \
--size-only
#--exclude '*/*'
<file_sep>/01_preproc.bash
#!/usr/bin/env bash
# 1. preproc mprage if needed
# 2. preproc functional
export AFNI_AUTOGZIP="YES"
export FSLOUTPUTTYPE="NIFTI_GZ"
# go to script directory
scriptdir=$(cd $(dirname $0); pwd)
cd $(dirname $0)
## what do the final files look like
# with these known, we can skip finished visits
# enabling quick resume
finalmprage=mprage_final.nii.gz
finalwarp=mprage_final_warpfield.nii.gz
finalrun=
# get MAXJOBS and WAITTIME.
# -- done this way, the settings can change mid run
settingsrc="settingsrc.sh"
source $settingsrc
function preprocess {
subjdir="$1"
## get subj/date as variables
subjdate=$(basename $subjdir)
subj=${subjdate%%_*}
vdate=${subjdate##*_}
echo "$subjdate $subj $vdate"
## PROCESS MPRAGE
# ... if needed
mpragedir="$(find $subjdir -type d -maxdepth 1 -name "axial_mprage*" | sed 1q)"
[ -z "$mpragedir" -o ! -d "$mpragedir" ] && echo "NO mpragedir in $subjdir" && return
mprage="$(find $subjdir -name "$finalmprage" | sed 1q)"
warprage="$(find $subjdir -name "$finalwarp" | sed 1q)"
# if we do not have warprage or mprage
if [ -z "$mprage" -o \
-z "$warprage" ]; then
cd $mpragedir
echo "T1 $subj $vdate"
preprocessMprage -d y -o mprage_final.nii.gz
cd -
fi
# test that mprage preprocessing worked
mprage="$(find $subjdir -name "$finalmprage" | sed 1q)"
warprage="$(find $subjdir -name "$finalwarp" | sed 1q)"
if [ -z "$mprage" -o \
-z "$warprage" ]; then
echo "failed to create $finalmprage and $finalwarp for visit $subjdate"
return
fi
## PREPROCESS FUNCTIONAL
# for each run of bars reward
for BarsRun in $subjdir/BarsRewards_AntiX4_384x384*; do
cd $BarsRun
echo "T2 $subj $vdate"
echo "preprocessFunctional $BarsRun"
cd -
done
}
function exiterror {
echo $@
exit 1;
}
## do not spam the system with jobs
# wait until less than max jobs are queued
function waitforjobs {
message="$@"
# make sure we have a MAXJOBS
[ -z "$settingsrc" -o ! -r "$settingsrc" ] && exiterror "cannot find a settingsrc file"
source $settingsrc
[ -z "$MAXJOBS" -o -z "$WAITTIME" ] && exiterror "cannot read MAXJOBS or WAITITME form $settingrc file"
# wait until we clear below MAXJOBS
njobs=$(jobs -p | wc -l)
while [[ "$njobs" -ge "$MAXJOBS" ]]; do
echo
echo "have ${njobs// } jobs, waiting $WAITTIME"
jobs | sed 's/^/ /'
echo $message
echo
sleep $WAITTIME;
njobs=$(jobs -p | wc -l)
done
}
waitforjobs # no jobs to wait for, but makes sure settings are correct
for subjdir in $scriptdir/subjs/1*_*/; do
preprocess $subjdir &
waitforjobs "last visit submitted: $subjdir"
done
wait
#mail
| 6d6253a5806bd3d65789fcc56ef226846c0be77f | [
"Shell"
] | 2 | Shell | LabNeuroCogDevel/BarsBTC | 21448539c173ce095bc4d2f38242dc0ed177554a | c5f0ee12ac2d862747ffd61ccb6c9af8922fbadd | |
refs/heads/master | <file_sep># ai-car-plate
ai-car-plate
<file_sep>import numpy as np
import paddle.fluid as fluid
import matplotlib.pyplot as plt
import argparse
from PIL import Image
from plate_utils import plate_split
from lenet import LeNet5
from gen_utils import gen_dataset
def parse_args():
parser = argparse.ArgumentParser("Prediction Parameters")
parser.add_argument(
'--weight_file',
type=str,
default='car_plate_10',
help='the path of model parameters')
parser.add_argument(
'--test_plate',
type=str,
default='test/001.png',
help='the test plate')
args = parser.parse_args()
return args
args = parse_args()
WEIGHT_FILE = args.weight_file
TEST_PLATE = args.test_plate
def predict(model, params_file_path, test_plate):
with fluid.dygraph.guard():
model_state_dict, _ = fluid.load_dygraph(params_file_path)
model.load_dict(model_state_dict)
model.eval()
_, _, character_mapper = gen_dataset()
img_character_dict = plate_split(test_plate)
plate = []
for key in img_character_dict:
img = img_character_dict[key]
img = img.flatten().astype('float32') / 255.0
img = img.reshape(1, 1, 20, 20)
img = fluid.dygraph.to_variable(img)
logits = model(img)
result = fluid.layers.softmax(logits).numpy()
result = np.argmax(result[0])
result = character_mapper.get(result, '')
plate.append(result)
print("key:{}, result:{}".format(key, result))
plt.imshow(Image.open(test_plate))
print("\n车牌识别结果为:", end='')
for i in range(len(plate)):
print(plate[i], end='')
print("\n")
plt.show()
if __name__ == '__main__':
test_plate = TEST_PLATE
params_file_path = WEIGHT_FILE
with fluid.dygraph.guard():
model = LeNet5('LeNet5', num_classes=65)
predict(model, params_file_path, test_plate)
<file_sep>import numpy as np
import cv2
from gen_utils import gen_dataset
def img_mapper(sample):
img, label = sample
img = cv2.imdecode(np.fromfile(img, dtype=np.uint8), 0)
img = img.flatten().astype('float32') / 255.0
img = img.reshape(1, 20, 20)
return img, label
def data_loader(img_list, batch_size=100, mode='train'):
def reader():
if mode == 'train':
np.random.shuffle(img_list)
batch_imgs = []
batch_labels = []
for info in img_list:
path, label = info
img, label = img_mapper((path, label))
batch_imgs.append(img)
batch_labels.append(label)
if len(batch_imgs) == batch_size:
img_array = np.array(batch_imgs).astype('float32')
labels_array = np.array(batch_labels).astype('int64').reshape(-1, 1)
yield img_array, labels_array
batch_imgs = []
batch_labels = []
if len(batch_imgs) > 0:
img_array = np.array(batch_imgs).astype('float32')
labels_array = np.array(batch_labels).astype('int64').reshape(-1, 1)
yield img_array, labels_array
return reader
if __name__ == '__main__':
train_dataset, _, _ = gen_dataset()
train_loader = data_loader(train_dataset)
train_img, train_label = next(train_loader())
print(train_img.shape)
print(train_label.shape)
<file_sep>import paddle.fluid as fluid
from paddle.fluid.dygraph.nn import Conv2D, Pool2D, FC
class LeNet5(fluid.dygraph.Layer):
def __init__(self, name_scope, num_classes):
super(LeNet5, self).__init__(name_scope)
self.conv1 = Conv2D(self.full_name(), num_filters=50, filter_size=5, stride=1)
self.pool1 = Pool2D(self.full_name(), pool_size=2, pool_stride=1, pool_type='max')
self.conv2 = Conv2D(self.full_name(), num_filters=32, filter_size=3, stride=1)
self.pool2 = Pool2D(self.full_name(), pool_size=2, pool_stride=1, pool_type='max')
self.fc1 = FC(self.full_name(), size=num_classes, act='softmax')
def forward(self, x):
x = self.conv1(x)
x = self.pool1(x)
x = self.conv2(x)
x = self.pool2(x)
x = self.fc1(x)
return x
<file_sep>import numpy as np
import cv2
def plate_split(plate_file):
img_character_dict = {}
img_plate = cv2.imread(plate_file)
img_plate = cv2.cvtColor(img_plate, cv2.COLOR_RGB2GRAY)
th, binary_plate = cv2.threshold(img_plate, 175, 255, cv2.THRESH_BINARY)
result = []
for col in range(binary_plate.shape[1]):
result.append(0)
for row in range(binary_plate.shape[0]):
result[col] = result[col] + binary_plate[row][col] / 255
character_dict = {}
num = 0
i = 0
while i < len(result):
if result[i] == 0:
i += 1
else:
index = i + 1
while result[index] != 0:
index += 1
character_dict[num] = [i, index - 1]
num += 1
i = index
for i in range(8):
if i == 2:
continue
padding = (170 - (character_dict[i][1] - character_dict[i][0])) / 2
img_character = np.pad(binary_plate[:, character_dict[i][0]:character_dict[i][1]],
((0, 0), (int(padding), int(padding))), 'constant', constant_values=(0, 0))
img_character = cv2.resize(img_character, (20, 20))
# cv2.imwrite(str(i) + '.png', img_character)
img_character_dict[i] = img_character
return img_character_dict
if __name__ == '__main__':
img_character_dict = plate_split('test/001.png')
for key in img_character_dict:
print("key:{}, img shape:{}".format(key, img_character_dict[key].shape))
<file_sep>import os
folder_mapper = {'0': '0',
'1': '1',
'2': '2',
'3': '3',
'4': '4',
'5': '5',
'6': '6',
'7': '7',
'8': '8',
'9': '9',
'A': 'A',
'B': 'B',
'C': 'C',
'cuan': '川',
'D': 'D',
'E': 'E',
'e1': '鄂',
'F': 'F',
'G': 'G',
'gan': '赣',
'gan1': '甘',
'gui': '贵',
'gui1': '桂',
'H': 'H',
'hei': '黑',
'hu': '沪',
'J': 'J',
'ji': '冀',
'ji1': '吉',
'jin': '津',
'jing': '京',
'K': 'K',
'L': 'L',
'liao': '辽',
'lu': '鲁',
'M': 'M',
'meng': '蒙',
'min': '闽',
'N': 'N',
'ning': '宁',
'P': 'P',
'Q': 'Q',
'qing': '青',
'qiong': '琼',
'R': 'R',
'S': 'S',
'shan': '陕',
'su': '苏',
'sx': '晋',
'T': 'T',
'U': 'U',
'V': 'V',
'W': 'W',
'wan': '皖',
'X': 'X',
'xiang': '湘',
'xin': '新',
'Y': 'Y',
'yu': '豫',
'yu1': '渝',
'yue': '粤',
'yun': '云',
'Z': 'Z',
'zang': '藏',
'zhe': '浙'}
# 生成车牌字符图像列表
def gen_dataset():
data_path = 'train'
character_folders = os.listdir(data_path)
label = 0
train_dataset = []
valid_dataset = []
character_mapper = {}
for character_folder in character_folders:
if character_folder == '.DS_Store' \
or character_folder == '.ipynb_checkpoints':
continue
character_mapper[label] = folder_mapper.get(character_folder, '')
# print(str(label) + " " + character_folder + " " + character_mapper[label])
character_imgs = os.listdir(os.path.join(data_path, character_folder))
for i in range(len(character_imgs)):
if i % 10 == 0:
valid_dataset.append((os.path.join(os.path.join(data_path, character_folder),
character_imgs[i]), label))
else:
train_dataset.append((os.path.join(os.path.join(data_path, character_folder),
character_imgs[i]), label))
label = label + 1
return train_dataset, valid_dataset, character_mapper
if __name__ == '__main__':
train_dataset, valid_dataset, character_mapper = gen_dataset()
print('train size:{}, valid_size:{}, mapper_len:{}'.format(len(train_dataset),
len(valid_dataset),
len(character_mapper)))
<file_sep>import matplotlib.pyplot as plt
# 绘制训练趋势图
def draw_figure(acc, val_acc, loss, val_loss):
count = len(acc)
epochs = range(1, count + 1)
plt.plot(epochs, acc, 'bo', label='Training accuracy')
plt.plot(epochs, val_acc, 'b', label='Validation accuracy')
plt.title('Training and validation accuracy')
plt.legend()
plt.savefig('car_plate_acc_{}.png'.format(count))
plt.figure()
plt.plot(epochs, loss, 'bo', label='Training loss')
plt.plot(epochs, val_loss, 'b', label='Validation loss')
plt.title('Training and validation loss')
plt.legend()
plt.savefig('car_plate_loss_{}.png'.format(count))
# plt.show()
<file_sep>certifi==2019.11.28
chardet==3.0.4
cycler==0.10.0
decorator==4.4.1
funcsigs==1.0.2
graphviz==0.13.2
idna==2.9
kiwisolver==1.1.0
matplotlib==3.1.3
nltk==3.4.5
numpy==1.18.1
objgraph==3.4.1
opencv-python==4.2.0.32
paddlepaddle==1.6.3
Pillow==7.0.0
prettytable==0.7.2
protobuf==3.11.3
pyparsing==2.4.6
python-dateutil==2.8.1
PyYAML==5.3
rarfile==3.1
requests==2.23.0
scipy==1.4.1
six==1.14.0
urllib3==1.25.8
<file_sep>import datetime
import paddle.fluid as fluid
import numpy as np
import argparse
from reader import data_loader
from lenet import LeNet5
from figure_utils import draw_figure
from gen_utils import gen_dataset
def parse_args():
parser = argparse.ArgumentParser("Training Parameters")
parser.add_argument(
'--num_epoch',
type=int,
default=20,
help='the epoch num')
parser.add_argument(
'--batch_size',
type=int,
default=100,
help='the batch size')
args = parser.parse_args()
return args
args = parse_args()
NUM_EPOCH = args.num_epoch
BATCH_SIZE = args.batch_size
def train(model):
with fluid.dygraph.guard():
print('start training ... ')
begin = datetime.datetime.now()
model.train()
epoch_num = NUM_EPOCH
all_acc = []
all_val_acc = []
all_loss = []
all_val_loss = []
opt = fluid.optimizer.Momentum(learning_rate=0.001, momentum=0.9)
train_dataset, valid_dataset, _ = gen_dataset()
train_loader = data_loader(train_dataset, batch_size=BATCH_SIZE, mode='train')
valid_loader = data_loader(valid_dataset, batch_size=BATCH_SIZE, mode='valid')
for epoch in range(epoch_num):
accuracies = []
losses = []
for batch_id, data in enumerate(train_loader()):
x_data, y_data = data
img = fluid.dygraph.to_variable(x_data)
label = fluid.dygraph.to_variable(y_data)
logits = model(img)
cost = fluid.layers.cross_entropy(input=logits, label=label)
acc = fluid.layers.accuracy(input=logits, label=label)
avg_cost = fluid.layers.mean(cost)
if batch_id % 50 == 0:
print("epoch: {}, batch_id: {}, acc is {}, loss is: {}".format(epoch,
batch_id,
acc.numpy(),
avg_cost.numpy()))
# 反向传播,更新权重,清除梯度
avg_cost.backward()
opt.minimize(avg_cost)
model.clear_gradients()
accuracies.append(acc.numpy())
losses.append(avg_cost.numpy())
print("[train] accuracy/loss: {}/{}".format(np.mean(accuracies), np.mean(losses)))
all_acc.append(np.mean(accuracies))
all_loss.append(np.mean(losses))
# 每5轮过后保存模型参数
if (epoch % 5 == 0) or (epoch == epoch_num - 1):
fluid.save_dygraph(model.state_dict(), 'car_plate_{}'.format(epoch))
model.eval()
accuracies = []
losses = []
for batch_id, data in enumerate(valid_loader()):
x_data, y_data = data
img = fluid.dygraph.to_variable(x_data)
label = fluid.dygraph.to_variable(y_data)
logits = model(img)
cost = fluid.layers.cross_entropy(input=logits, label=label)
acc = fluid.layers.accuracy(input=logits, label=label)
avg_cost = fluid.layers.mean(cost)
accuracies.append(acc.numpy())
losses.append(avg_cost.numpy())
print("[validation] accuracy/loss: {}/{}".format(np.mean(accuracies), np.mean(losses)))
all_val_acc.append(np.mean(accuracies))
all_val_loss.append(np.mean(losses))
# 绘图,每5轮绘制一个趋势图
if (epoch % 5 == 0) or (epoch == epoch_num - 1):
count = len(all_acc)
if count > 2:
sub_all_acc = all_acc[2:]
sub_all_val_acc = all_val_acc[2:]
sub_all_loss = all_loss[2:]
sub_all_val_loss = all_val_loss[2:]
draw_figure(sub_all_acc, sub_all_val_acc, sub_all_loss, sub_all_val_loss)
model.train()
end = datetime.datetime.now()
seconds = (end - begin).seconds
print("finished. total cost {}".format(datetime.timedelta(seconds=seconds)))
if __name__ == '__main__':
with fluid.dygraph.guard():
model = LeNet5('LeNet5', num_classes=65)
train(model)
| 31a7fa3be0d73472e0f206e3ebb31e1aeb3bd002 | [
"Markdown",
"Python",
"Text"
] | 9 | Markdown | chenmj201601/ai-car-plate | 6235d24caeea4fbe915cff49c095c155497a25a0 | bea1e0a46aee08969db5049a39a01e03d5ccd967 | |
refs/heads/master | <file_sep>
# My typing
Files related to mechanical keyboards and typing.
# Keyboard
Layouts made using [keyboard-layout-editor.com](http://www.keyboard-layout-editor.com/).
## Current keyboard: FILCO, Majestouch-2
FILCO, Majestouch-2, tenkeyless (88 keys) and fullsize (105 keys), ISO Danish layout
Replaced the controllers with The Pegasus Hoof (on tenkeyless) and Tiger Lily (on fullsize) controllers from http://bathroomepiphanies.com/controllers/

# My Typeracer statistics


<file_sep>#!/usr/bin/env python2
# Get, store, and visualize Typeracer results
import json
import matplotlib.pyplot as plt
import numpy as np
import os
import requests
import shutil
import sys
import time
def moving_average(a, n=3):
ret = np.cumsum(a, dtype=float)
ret[n:] = ret[n:] - ret[:-n]
return ret[n - 1:] / n
# Get data
url = "http://data.typeracer.com/games?playerId=tr:skrivemaskinen&n=999999999"
r = requests.get(url)
status_code = r.status_code
if status_code == 404:
print('ERROR: status code: %s Not Found' % status_code)
sys.exit(1)
content = r.content
# Store data
outfile = 'data/data.json'
f = open(outfile, 'w')
f.write(content)
f.close()
# Compute statistics
with open(outfile) as datafile:
data = json.load(datafile)
# Words per minute
wpm = [d['wpm'] for d in data]
wpm = np.asarray(list(reversed(wpm)))
window_size = 5
wpm_moving_avg = moving_average(wpm, n=window_size)
recent_mean = np.mean(wpm[-10:])
outplotfile = 'plot/newest.png'
fig, ax = plt.subplots(nrows=1, ncols=1)
today = time.strftime("%Y%m%d")
plt.title("Typing Speed in TyperRacer, %s (Recent avg.=%i)" \
% (today, recent_mean), fontsize=13)
plt.xlabel("\nRace no.", fontsize=10)
plt.ylabel("Words per minute (5 chars/word)", fontsize=10)
plt.plot(wpm, color='#cccccc')
plt.plot(wpm_moving_avg, color='#000000', \
label='Moving avg. (n=%s)' % window_size)
plt.legend(prop={'size': 9})
fig.savefig(outplotfile)
plt.close(fig)
| 159dae4c4e3a27df3f3e423457da4f448e368b0b | [
"Markdown",
"Python"
] | 2 | Markdown | thomasboevith/mytyping | 7c174a2031436b6f0c1b831e024c660b4e0f8814 | 4c9834934b46128f841d03870edc9d5c3a10a2e3 | |
refs/heads/master | <repo_name>F1703/proyecto-b<file_sep>/app/Http/Controllers/MateriaController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests;
use App\Materia;
use Laracasts\Flash\Flash;
class MateriaController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
//
$materias=Materia::all();
return view('materia.index')
->with('materias', $materias);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
return view('materia.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$mat= new Materia($request->all());
$mat->save();
Flash::success('La materia '.$mat->nombre.' se creo con exito!!');
return redirect()->route('materia.index');
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
<file_sep>/app/Comentario.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Comentario extends Model
{
//
protected $table = 'comentarios';
protected $fillable =['contenido','user_id','publicacion_id'];
public function publicacion(){
return $this->hasMany('App\Publicacion','publicacion_id','user_id');
}
public function user(){
return $this->belongsTo('App\User');
}
public function scopeSearch($query,$id){
return $query->where('publicacion_id','=',$id);
}
}
<file_sep>/app/Http/Controllers/PublicacionController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests;
use App\Categoria;
use App\Materia;
use App\Publicacion;
use App\Archivo;
use Laracasts\Flash\Flash;
class PublicacionController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
//
$posts = Publicacion::orderby('id','ASC')->paginate(3);
// $categorias=Categoria::all();
// $materias=Materia::all();
return view('post.index')
->with('posts', $posts);
// ->with('categorias', $categorias)
// ->with('materias', $materias);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
<file_sep>/app/Http/Controllers/PostController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests;
use App\Categoria;
use App\Materia;
use App\Publicacion;
use App\Archivo;
use App\Comentario;
use Laracasts\Flash\Flash;
class PostController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
//
$posts = Publicacion::searchUser(\Auth::user()->id)->orderby('id','asc')->paginate(2);
return view('post.index')
->with('posts', $posts);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
$categorias=Categoria::lists('nombre','id');
$materias = Materia::lists('nombre','id');
return view('post.create')
->with('categorias', $categorias)
->with('materias', $materias);
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//
// dd($request->all());
if ($request->file('archivo_id') !=null) {
$file=$request->file('archivo_id');
$name= date('d.m.Y').'_'.time().'_'.$file->getClientOriginalName().'_.'.$file->getClientOriginalExtension();
$path=public_path().'/files';
// $file->move($path,$name);
$archivo= new Archivo;
$archivo->nombre= $name;
$archivo->save();
$publicacion=new Publicacion($request->all());
$archivo= Archivo::find($archivo->id);
$publicacion->archivo_id=$archivo->id;
$publicacion->save();
}else {
$publicacion=new Publicacion($request->all());
$publicacion->save();
}
Flash::success('Se creo con exito el usario '.$publicacion->slug.' !!');
return redirect()->route('post.create');
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($slug)
{
//
;
$post= Publicacion::Search($slug)->get();
$post->each(function($post){
// $comentario= Comentario::Search($post->id)->get();
// $comentario->publicacion;
// dd($comentario);
$post->comentario;
});
return view('post.show')
->with('post', $post);
// ->with('comentario', $comentario);
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
<file_sep>/app/Materia.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Materia extends Model
{
//
protected $table = "materias";
protected $fillable =['nombre'];
public function publicacion(){
return $this->hasOne('App\Publicacion');
}
}
<file_sep>/app/Publicacion.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
use Cviebrock\EloquentSluggable\Sluggable;
class Publicacion extends Model
{
//
use Sluggable;
protected $table = 'publicacions';
protected $fillable =['titulo','intro','cuerpo','slug','user_id','categoria_id','materia_id','archivo_id'];
public function sluggable()
{
return [
'slug' => [
'source' => 'titulo'
]
];
}
public function archivo(){
return $this->belongsTo('App\Archivo');
}
public function materia(){
return $this->belongsTo('App\Materia');
}
public function categoria(){
return $this->belongsTo('App\Categoria');
}
public function user(){
return $this->belongsTo('App\User');
}
public function comentario(){
return $this->hasMany('App\Comentario');
}
public function scopeSearch($query, $slug){
return $query->where('slug','=',$slug);
}
public function scopeSearchUser($query,$user_id){
return $query->where('user_id','=', $user_id);
}
}
| 6c02abbc6cafd07c46eaf9bba913852861d4156f | [
"PHP"
] | 6 | PHP | F1703/proyecto-b | 636e9e26ec1147db20deed3466c7bd00a8cd075a | b20e911b73e5a008daf2280102296a6fe75a1a22 | |
refs/heads/master | <file_sep>from jinja2 import Environment
import mycsv
header, data = mycsv.readcsv(mycsv.getdata())
XML = """<?xml version="1.0"?>
<file>
<headers>{% for name in header %}{{name}}{% if name != header[-1] %},{% endif %}{% endfor%}</headers>
<data>{% for list in data %}
<record>
{% for item in list -%}
<{{header[loop.index0].replace(" ", "_")}}>{{item}}</{{header[loop.index0].replace(" ", "_")}}>
{%- endfor %}
</record>{% endfor %}
</data>
</file>"""
print Environment().from_string(XML).render(header=header, data=data)<file_sep>"""
Function that finds the best fit line in the sense of least squares
to a set of data consisting of paired observations in the form (x, y).
"""
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
def leastSquares(filename):
#read in only columns 'x' and 'y'
fields = ['x', 'y']
fr = pd.read_csv(filename, usecols=fields)
col1 = np.array(fr.x, dtype=float).reshape(len(fr.x), 1)
col2 = np.ones(len(fr.x), dtype=float).reshape(len(fr.x), 1)
b = np.array(fr.y, dtype=float).reshape(len(fr.y), 1)
matrixA = np.concatenate((col1, col2), axis=1)
Atranspose = np.transpose(matrixA)
At_A = np.dot(Atranspose, matrixA)
At_b = np.dot(Atranspose, b)
xhat = np.linalg.solve(At_A, At_b)
slope = xhat.item(0)
intercept = xhat.item(1)
print
print "The slope of the " + filename + " data is..."
print slope
print "The intercept of the " + filename + " data is..."
print intercept
A_xhat = np.dot(matrixA, xhat)
minimize = A_xhat - b
square_minimize = minimize**2
sum_square = np.sum(square_minimize)
print "The sum of the squares of the distances for the " + filename + " data is..."
print sum_square
line = slope * col1 + intercept
plt.plot(fr.x, fr.y, '*')
plt.plot(fr.x, line)
plt.show()
<file_sep>from jinja2 import Environment
import mycsv
header, data = mycsv.readcsv(mycsv.getdata())
HTML = """<html>
<body>
<table>
<tr>{% for item in header %}<th>{{item}}</th>{% endfor %}</tr>{% for list in data %}
<tr>{% for item in list %}<td>{{item}}</td>{% endfor %}</tr>{% endfor %}
</table>
</body>
</html>"""
print Environment().from_string(HTML).render(header=header, data=data)<file_sep>import numpy as np
"""
Define a 10x10 matrix A whose entries are the numbers from 1 to 100 ordered consecutively going across rows
(so a_1,1 = 1, a_2,1 = 11, a_10,1 = 91, etc)
Let v = (1, 2, ...10), and b = (1, 1, ...1).
Compute Av and solve Ax = b and Ax = v using linalg.solve.
Verify your solution by computing Ax.
"""
# 10 by 10 matrix A from 1 to 100 (Note: This is a Singular Matrix!)
A = np.arange(1,101).reshape(10,10)
# 10 by 1 vector from 1 to 10
v = np.arange(1,11).reshape(10,1)
# 10 by 1 vector, all ones
b = np.ones((10,), dtype=np.int).reshape(10,1)
# checking...
# print A
# print v
# print b
# compute Av
print "Computing Av..."
print np.dot(A, v)
# solve for Ax = b
# ERROR: matrix A is a Singular matrix, so x cannot be solved.
# Thus, the next two lines of code are commented out.
# x = np.linalg.solve(A, b)
# print x
# solve for Ax = v
# ERROR: matrix A is still a Singular matrix, so x cannot be solved.
# Thus, the next two lines of code are commented out.
# x2 = np.linalg.solve(A, v)
# print x2
# verify with Ax
# ERROR: cannot verify with Ax because x is still not solved.
# Thus, the next line of code is commented out.
# print np.dot(A, x)
"""
How do we solve this? Add some noise to the matrix!
"""
"""
Use the rand command to build a 10x10 matrix R(e).
Verify now that you can compute the questions above for various values of 1 >> e > 0 for the new matrix A + R(e).
"""
# epsilon e
e = np.random.random_sample()
print "The epilson is: " + str(e)
# 10 by 10 matrix R(e)
Re = np.random.uniform(-e, e, size=(10, 10))
# 10 by 10 matrix A from 1 to 100 (NOTE: This is a Singular Matrix!)
A = np.arange(1, 101).reshape(10, 10)
# 10 by 1 vector v from 1 to 10
v = np.arange(1, 11).reshape(10, 1)
# 10 by 1 vector b filled with all ones
b = np.ones((10,), dtype=np.int).reshape(10, 1)
# new 10 by 10 matrix Noise or N = A + Re
N = A + Re
print "The new matrix N = A + Re is..."
print N
# solve for Nx = b using linalg.solve
x = np.linalg.solve(N,b)
print "Solution: using linalg.solve, vector x for Nx = b is..."
print x
# solve for Nx = v using linalg.solve
x2 = np.linalg.solve(N,v)
print "Solution: using linalg.solve, vector x2 for Nx = v is..."
print x2
# verify solution for x is correct
# should print vector b
print "Verifying the solution for x..."
print np.dot(N,x)
# verify solution for x2 is correct
# should print vector v
print "Verifying the solution for x2..."
print np.dot(N,x2)
<file_sep># USF MS in Data Science & Analytics Coursework
### Personal Codes & Lecture Notes
NOTE: Please note that not all of my notes and codes are up yet, but my goal is to work on getting all my stuff up and running by spending at least 5-10minutes every day updating my GitHub (: Thanks!
### 1. [Linear Algebra](https://github.com/t2liu/usf_personal/tree/master/linearalgebra) -
- **[Solving Linear Matrix Equations](https://github.com/t2liu/usf_personal/blob/master/linearalgebra/linalg.solve.py)**: how to use the `linalg.solve` function from NumPy
- **[Eliminate.py](ADD LINK)**
- **[leastSquares.py](https://github.com/t2liu/usf_personal/blob/master/linearalgebra/leastSquares.py)**: function that finds the best fit line in the sense of least squares to a set of data consisting of paired observations in the form (x, y)
### 2. [Data Translation Pipeline]() -
### 3. [Distributed Data Systems](https://github.com/t2liu/usf_personal/tree/master/distributeddatasystems) -
- **[Lecture Notes Day 1](https://github.com/t2liu/usf_personal/blob/master/distributeddatasystems/Lecture%20Notes%20Day%201-%20Distributed%20Data%20Systems.ipynb)**: notes from Day 1 of Distributed Data Systems course
### 4.
### 5.
### 6.
<file_sep>from jinja2 import Environment
import mycsv
header, data = mycsv.readcsv(mycsv.getdata())
header2 = ['"' + name + '"' for name in header]
rows = len(data)
cols = len(header)
JSON = """{
"headers":[{% for name in header %}"{{name}}"{% if name != header[-1] %}, {% endif %}{% endfor %}],
"data":[{% for i in range(rows) %}
{
{% for j in range(cols) %}{{header2[j]}}:"{{data[i][j]}}"{% if j != cols-1 %}, {% endif %}{% endfor %}
}{% if i != rows-1 %},{% endif %}{% endfor %}
]
}"""
print Environment().from_string(JSON).render(header=header, data=data, header2=header2, rows=rows, cols=cols) | b41ef996d7af5e5b4fb5f2531557a20499c837e8 | [
"Markdown",
"Python"
] | 6 | Python | t2liu/usf_personal | fa07453e4c01dbc8cd809fd22ab494205598d68b | 6ca27443b5bdc612ddd5b2dbc4fbdda0bf46a2c3 | |
refs/heads/master | <file_sep>$(document).ready(function() {
var changePassword = $('#change_password'),
activate = $('#activate_account'),
changeDialog = $('#change_dialog'),
activateDialog = $('#activate_dialog');
activateDialog.dialog({
autoOpen : false,
buttons: [
{
text: "Accept",
click: function() {
activateAccount(this);
}
},
{
text: "Cancel",
click: function() {
$( this ).dialog( "close" );
}
}
],
height: 500,
width: 600,
modal: true,
position: "center",
resizable: false
});
if(activate.length > 0){
activate.on('click', function(){
activateDialog.dialog("open");
});
}
changeDialog.dialog({
autoOpen : false,
buttons: [
{
text: "Submit",
click: function() {
submitChangePassword(this);
}
},
{
text: "Cancel",
click: function() {
$( this ).dialog( "close" );
}
}
],
height: 500,
width: 600,
modal: true,
position: "center",
resizable: false
});
changePassword.on('click', function(){
changeDialog.dialog("open");
});
function submitChangePassword(dialog){
var old = $(dialog).find("#old_password"),
newP = $(dialog).find("#new_password"),
confirm = $(dialog).find("#confirm_new_password");
if(newP.val() !== confirm.val()){
alert('passwords do not match!');
return;
}else if(newP.val().length < 6){
alert('passwords must be a minimum of 6 characters');
return;
}
$.ajax({
dataType: 'json',
type: 'POST',
url: '/api/user/changePassword',
data: JSON.stringify({
oldPassword : old.val(),
newPassword : <PASSWORD>()
}),
success:function(data){
if(data.message == "success"){
alert('Password successfully changed!');
}else if(data.message == "incorrect"){
alert('Your old password was wrong');
}else{
alert('Something went wrong');
}
$(dialog).dialog('close');
},
error:function(){
}
});
}
function activateAccount(dialog){
$.ajax({
dataType: 'json',
type: 'POST',
url: '/api/user/activate',
success:function(data){
$('#activate_container').html('Your Account is Active');
$(dialog).dialog("close");
},
error:function(){
}
});
}
});<file_sep>SMS Journal
=======================
Introduction
------------
This is a class project. I'm using ZF2, mongoDB, doctrine 2 ODM, and Nexmo to complete everything.
<file_sep><?php
namespace Application\Controller;
use Zend\Mvc\Controller\AbstractRestfulController;
use Zend\View\Model\JsonModel;
use Zend\EventManager\EventManagerInterface;
use Zend\Json\Json;
use Application\Model\UserModel;
class BaseRestfulController extends AbstractRestfulController
{
/**
* @var Doctrine\ODM\MongoDB\DocumentManager
*/
protected $dm;
/**
* @var Application\Mapper\Manager
*/
protected $mManager;
public function getDocumentManager() {
if (null === $this->dm) {
$this->dm = $this->getServiceLocator()->get('doctrine.documentmanager.odm_default');
}
return $this->dm;
}
public function getAuthenticatedUser() {
$user = $this->zfcUserAuthentication()->getAuthService()->getIdentity();
if(!$user){
return $this->redirect()->toRoute('application', array('controller'=> 'index', 'action' => 'logout'));
}
return $user;
}
public function hasIdentity() {
$user = $this->zfcUserAuthentication()->getAuthService()->getIdentity();
if(!$user){
return false;
}
return true;
}
}
<file_sep><?php
namespace Application\Controller;
use Zend\Mvc\Controller\AbstractRestfulController;
use Zend\View\Model\JsonModel;
use Zend\EventManager\EventManagerInterface;
class ApiController extends AbstractRestfulController
{
protected $collectionOptions = array('GET', 'POST');
protected $resourceOptions = array('GET', 'PUT', 'DELETE');
protected function _getOptions()
{
if($this->params()->fromRoute('id', false)) {
return $this->resourceOptions;
}
return $this->collectionOptions;
}
public function options()
{
$response = $this->getResponse();
$response->getHeaders()
->addHeaderLine('Allow', implode(',', $this->_getOptions()));
return $response;
}
public function setEventManager(EventManagerInterface $events) {
$this->events = $events;
$events->attach('dispatch', array($this, 'checkOptions'), 10);
}
public function checkOptions($e){
if(in_array($e->getResopnse()->getMethod(), $this->_getOptions())){
return;
}
$response = $this->getResponse();
$response->setStatusCode(405);
return $response;
}
/**
* Create a new resource
*
* @param mixed $data
* @return mixed
*/
public function create($data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Delete an existing resource
*
* @param mixed $id
* @return mixed
*/
public function delete($id)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Delete the entire resource collection
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.1.0); instead, raises an exception if not implemented.
*
* @return mixed
*/
public function deleteList()
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Return single resource
*
* @param mixed $id
* @return mixed
*/
public function get($id)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Return list of resources
*
* @return mixed
*/
public function getList()
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Retrieve HEAD metadata for the resource
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.1.0); instead, raises an exception if not implemented.
*
* @param null|mixed $id
* @return mixed
*/
public function head($id = null)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Respond to the PATCH method
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.1.0); instead, raises an exception if not implemented.
*
* @param $id
* @param $data
*/
public function patch($id, $data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Replace an entire resource collection
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.1.0); instead, raises an exception if not implemented.
*
* @param mixed $data
* @return mixed
*/
public function replaceList($data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Modify a resource collection without completely replacing it
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.2.0); instead, raises an exception if not implemented.
*
* @param mixed $data
* @return mixed
*/
public function patchList($data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Update an existing resource
*
* @param mixed $id
* @param mixed $data
* @return mixed
*/
public function update($id, $data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
}
<file_sep>App.views.register = Backbone.View.extend({
el: '#register_form',
events: {
"click #register_submit" : "submitHandler"
},
submitHandler: function(){
}
});<file_sep><?php
/**
* Zend Framework (http://framework.zend.com/)
*
* @link http://github.com/zendframework/ZendSkeletonApplication for the canonical source repository
* @copyright Copyright (c) 2005-2014 Zend Technologies USA Inc. (http://www.zend.com)
* @license http://framework.zend.com/license/new-bsd New BSD License
*/
namespace Application\Controller;
use Zend\Mvc\Controller\AbstractActionController;
use Zend\View\Model\ViewModel;
class IndexController extends AbstractActionController
{
public function indexAction()
{
return new ViewModel();
}
public function journalAction()
{
$user = $this->getAuthenticatedUser();
$this->layout()->setVariable("user", $user);
return new ViewModel();
}
public function activityAction()
{
$user = $this->getAuthenticatedUser();
$this->layout()->setVariable("user", $user);
return new ViewModel();
}
public function accountAction()
{
$user = $this->getAuthenticatedUser();
$this->layout()->setVariable("user", $user);
return new ViewModel();
}
public function registerAction()
{
return new ViewModel();
}
public function loginAction()
{
return $this->forward()->dispatch('zfcuser', array('action' => 'login'));
}
public function logoutAction()
{
$this->forward()->dispatch('zfcuser', array('action' => 'logout'));
}
public function getAuthenticatedUser() {
$user = $this->zfcUserAuthentication()->getAuthService()->getIdentity();
if(!$user){
return $this->redirect()->toRoute('application', array('controller'=> 'index', 'action' => 'logout'));
}
return $user;
}
}
<file_sep><?php
namespace Application\Repository;
use Doctrine\ODM\MongoDB\DocumentRepository,
Application\Model\UserModel;
class UserRepository extends DocumentRepository
{
public function create(UserModel $user)
{
$user->createdOn = $user->modifiedOn = time();
$user->status = "new";
$user = $this->encrypt($user);
$this->getDocumentManager()->persist($user);
return $user;
}
public function getActiveUsers()
{
$query = $this->getDocumentManager()->createQueryBuilder('Application\Model\UserModel')
->field('status')->equals('active');
$cursor = $query->getQuery()->execute();
$users = array();
foreach($cursor as $item){
array_push($users, $item);
}
return $users;
}
public function getByNumber($phoneNumber)
{
$user = $this->getDocumentManager()->createQueryBuilder('Application\Model\UserModel')
->field('phone_number')->equals($phoneNumber)
->getQuery()->getSingleResult();
return $user;
}
public function update(UserModel $user){
$user->modifiedOn = time();
return $user;
}
public function checkPasswordCustom($user, $old)
{
$bcrypt = new \Zend\Crypt\Password\Bcrypt();
$bcrypt->setCost(14);
$cryp = $bcrypt->create("password");
die();
$oldUser = new UserModel();
$oldUser->password = $old;
$oldUser = $this->encrypt($oldUser);
if($user->password == $oldUser->password){
return true;
}
return false;
}
public function encrypt(UserModel $user)
{
$bcrypt = new \Zend\Crypt\Password\Bcrypt();
$bcrypt->setCost(14);
$user->password = $bcrypt->create($user->password);
return $user;
}
}
<file_sep><?php
namespace Application\Controller;
use Zend\Mvc\Controller\AbstractRestfulController;
use Zend\View\Model\JsonModel;
use Zend\EventManager\EventManagerInterface;
use Zend\Json\Json;
use Application\Controller\BaseRestfulContoller;
use Application\Model\JournalModel;
class JournalController extends BaseRestfulController
{
public function createAction()
{
$user = $this->getAuthenticatedUser();
$rawdata = json_decode($this->getRequest()->getContent(), true);
$entry = new JournalModel();
$entry->type = $rawdata['type'];
$entry->entry = $rawdata['entry'];
$entry->fromSMS = $rawdata['fromSMS'];
$entry->fromSystem = $rawdata['fromSystem'];
$entry->timestamp = strtotime($rawdata['date'] ." ". $rawdata['time']);
$entry->user = $user;
$journalRepo = $this->getDocumentManager()->getRepository('Application\Model\JournalModel');
$newEntry = $journalRepo->create($entry);
$this->getDocumentManager()->flush();
$this->response->setStatusCode(201);
return new JsonModel(array('message'=>'msg', 'id' => $newEntry->id));
}
/**
* Return list of resources
*
* @return mixed
*/
public function getListAction()
{
$user = $this->getAuthenticatedUser();
$journalRepo = $this->getDocumentManager()->getRepository('Application\Model\JournalModel');
$journalEntries = $journalRepo->getByUser($user);
$journalArray = array();
foreach($journalEntries as $entry){
$time = explode(" ", $entry->timestamp->format('Y-m-d H:i:s'));
$ar = array(
"id" => $entry->id,
"type" => $entry->type,
"entry" => $entry->entry,
"date" => $time[0],
"time" => $time[1]
);
$journalArray[] = $ar;
}
$this->response->setStatusCode(200);
return new JsonModel($journalArray);
}
/**
* Delete an existing resource
*
* @param mixed $id
* @return mixed
*/
public function delete($id)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Delete the entire resource collection
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.1.0); instead, raises an exception if not implemented.
*
* @return mixed
*/
public function deleteList()
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Return single resource
*
* @param mixed $id
* @return mixed
*/
public function get($id)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Retrieve HEAD metadata for the resource
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.1.0); instead, raises an exception if not implemented.
*
* @param null|mixed $id
* @return mixed
*/
public function head($id = null)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Respond to the PATCH method
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.1.0); instead, raises an exception if not implemented.
*
* @param $id
* @param $data
*/
public function patch($id, $data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Replace an entire resource collection
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.1.0); instead, raises an exception if not implemented.
*
* @param mixed $data
* @return mixed
*/
public function replaceList($data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Modify a resource collection without completely replacing it
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.2.0); instead, raises an exception if not implemented.
*
* @param mixed $data
* @return mixed
*/
public function patchList($data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Update an existing resource
*
* @param mixed $id
* @param mixed $data
* @return mixed
*/
public function update($id, $data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
}
<file_sep><?php
namespace Application\Model;
use \Doctrine\ODM\MongoDB\Mapping\Annotations as ODM,
\Zend\InputFilter\Input,
\Zend\InputFilter\InputFilter,
\Zend\Validator,
\Zend\Filter,
Application\Model\BaseModel;
/**
* @ODM\Document(collection="users", repositoryClass="Application\Repository\UserRepository")
*
*/
class UserModel extends BaseModel implements \ZfcUser\Entity\UserInterface{
/**
* @ODM\Id
*/
protected $id;
/**
* @ODM\String
*/
protected $email;
/**
* @ODM\String
*/
protected $password;
/**
* @ODM\String
*/
protected $name;
/**
* @ODM\String
*/
protected $phone_number;
/**
* @ODM\String
*/
protected $status;
/**
* @ODM\Date
*/
protected $createdOn;
/**
* @ODM\Date
*/
protected $modifiedOn;
public function getProperties(){
return array("id", "email", "password", "name", "createdOn", "modifiedOn", "status");
}
/**
* Define the filters used to validate the user entity
* @return \Zend\InputFilter\InputFilter
*/
protected function getFilters(){
$inputFilter = new InputFilter();
$input = new Input('email');
$input->getValidatorChain()
->addValidator(new Validator\EmailAddress());
$inputFilter->add($input);
$input = new Input('password');
$input->getValidatorChain()
->addValidator(new Validator\StringLength(1));
$inputFilter->add($input);
return $inputFilter;
}
public function getDisplayName() {
return $this->name;
}
public function getEmail() {
return $this->email;
}
public function getId() {
return $this->id;
}
public function getPassword() {
return $this->password;
}
public function getState() {
return $this->status;
}
public function getUsername() {
return $this->name;
}
public function setDisplayName($displayName) {
$this->name = $displayName;
return $this;
}
public function setEmail($email) {
$this->email = $email;
return $this;
}
public function setId($id) {
$this->id = $id;
return $this;
}
public function setPassword($password) {
$this->password = $password;
return $this;
}
public function setState($state) {
return $this;
}
public function setUsername($username) {
$this->name = $username;
return $this;
}
}
<file_sep><?php
namespace Application\Repository;
use Doctrine\ODM\MongoDB\DocumentRepository,
Application\Model\JournalModel,
Application\Model\UserModel;
class JournalRepository extends DocumentRepository
{
public function create(JournalModel $entry)
{
$entry->createdOn = $entry->modifiedOn = time();
$this->getDocumentManager()->persist($entry);
return $entry;
}
public function getByUser(UserModel $user)
{
$query = $this->getDocumentManager()->createQueryBuilder('Application\Model\JournalModel')
->field('user')->references($user)
->field('fromSystem')->equals(false);
$cursor = $query->getQuery()->execute();
$entries = array();
foreach($cursor as $item){
array_push($entries, $item);
}
return $entries;
}
}
<file_sep>$(document).ready(function() {
var registerForm = $('#register_form'),
submitBtn = $('#register_submit');
submitBtn.on('click', function(event){
submitHandler();
});
function submitHandler(){
var user = registerForm.serializeObject(),
error = "";
user.phone_number = user.phone_number.replace(/[^0-9]/g, '');
if(!user.email.valueOf(user.confirm_email)){
error += "Emails do not Match! \n";
}
if(!user.password.valueOf(user.confirm_password)){
error += "Passwords do not Match! \n";
}
if(user.phone_number.length != 11){
error += "Phone number is not 11 digits! \n";
}
if(user.name.length <1){
error += "No name entered! \n";
}
if(error.length > 0){
alert(error);
return false;
}
$.ajax({
dataType: 'json',
type: 'POST',
url: '/api/user/create',
data: JSON.stringify(user),
success:function(data){
window.location.replace("/application/index/journal");
},
error:function(){
}
});
}
$.fn.serializeObject = function()
{
var o = {};
var a = this.serializeArray();
$.each(a, function() {
if (o[this.name] !== undefined) {
if (!o[this.name].push) {
o[this.name] = [o[this.name]];
}
o[this.name].push(this.value || '');
} else {
o[this.name] = this.value || '';
}
});
return o;
};
});
<file_sep>App.models.User = Backbone.Model.extend({
url: "/api/user"
});
<file_sep>$(document).ready(function() {
var datePicker = $('#date_select'),
dateImg = $('#date_img'),
addEntry = $('#add_entry'),
search = $('#search_journal'),
journalHeader = $('#entries_header'),
journal = $('#journal_entries'),
addDialog = $('#add_dialog'),
journalEntries;
addDialog.dialog({
autoOpen : false,
buttons: [
{
text: "Submit",
click: function() {
submitEntry(this);
}
},
{
text: "Cancel",
click: function() {
closeAddDialog(this);
}
}
],
height: 500,
width: 600,
modal: true,
position: "center",
resizable: false
});
Date.prototype.yyyymmdd = function() {
var yyyy = this.getFullYear().toString();
var mm = (this.getMonth()+1).toString(); // getMonth() is zero-based
var dd = this.getDate().toString();
return yyyy +"-"+ (mm[1]?mm:"0"+mm[0]) +"-"+ (dd[1]?dd:"0"+dd[0]); // padding
};
var d = new Date();
datePicker.attr('value', d.yyyymmdd());
datePicker.on('change', function(){
populateJournal()
});
addEntry.on('click', function(){
addDialog.dialog("open");
});
fetchEntries();
function submitEntry(dialog){
var type = $(dialog).find("#add_type"),
hour = $(dialog).find("#add_time_hour"),
minute = $(dialog).find("#add_time_minute"),
period = $(dialog).find("#add_time_period"),
entry = $(dialog).find("#add_entry_input");
var journalEntry = {
type : type.val(),
date : datePicker.val(),
time : hour.val() + ":" + minute.val() + " " + period.val(),
entry : entry.val(),
fromSMS : false,
fromSystem: false
};
$.ajax({
dataType: 'json',
type: 'POST',
url: '/api/journal/create',
data: JSON.stringify(journalEntry),
success:function(data){
location.reload();
},
error:function(){
}
});
}
function closeAddDialog(dialog){
$(dialog).dialog("close");
$(dialog).html($("#add_dialog_template").html());
}
function fetchEntries(){
$.ajax({
dataType: 'json',
type: 'GET',
url: '/api/journal/get-list',
success:function(data){
journalEntries = data;
populateJournal();
},
error:function(){
}
});
}
function populateJournal(){
journal.html($('#journal_header_template').html());
var currentDate = datePicker.val(),
todaysEntries = [],
i;
if(journalEntries.length > 0){
for(i in journalEntries){
if(journalEntries[i].date.valueOf() == currentDate.valueOf()){
todaysEntries.push(journalEntries[i]);
}
}
i = 0;
todaysEntries = todaysEntries.sort(function(a,b){
var bTime = b.time.split(":"),
aTime = a.time.split(":");
bTime = bTime[0] + bTime[1] + bTime[2];
aTime = aTime[0] + aTime[1] + aTime[2];
return aTime - bTime;
});
for(i in todaysEntries){
var html = $($(getTemplateByType(todaysEntries[i].type)).html());
html.find('.time').html(formatTime(todaysEntries[i].time));
html.find('.entry').html(todaysEntries[i].entry);
journal.append("<li class='"+getOddEven(parseInt(i)+1)+"'>"+html.html()+"</li>");
}
}else{
journal.append("<li class='even'>No Entries to Display</li>");
}
return;
}
function formatTime(time){
var timeAr = time.split(":"),
hour,
period = "PM";
if(parseInt(timeAr[0])<12){
period = "AM";
}
if(timeAr[0].valueOf() == "00"){
hour = "12";
}else if(parseInt(timeAr[0]) > 12){
hour = parseInt(timeAr[0]) - 12;
}else{
hour = parseInt(timeAr[0]);
}
return hour + ":" + timeAr[1] + " " + period;
}
function getOddEven(num) {
if((num % 2) == 1){
return "even";
}else{
return "odd";
}
}
function getTemplateByType(type){
var id = "";
switch (type) {
case "activity":
id = "run_entry_template";
break;
case "mood":
id = "mood_entry_template";
break;
case "food":
id = "food_entry_template";
break;
case "misc":
id = "misc_entry_template";
break;
case "sleep":
id = "sleep_entry_template";
break;
case "update":
id = "update_entry_template";
break;
}
return "#" + id;
}
$.fn.serializeObject = function()
{
var o = {};
var a = this.serializeArray();
$.each(a, function() {
if (o[this.name] !== undefined) {
if (!o[this.name].push) {
o[this.name] = [o[this.name]];
}
o[this.name].push(this.value || '');
} else {
o[this.name] = this.value || '';
}
});
return o;
};
});<file_sep>var AppRouter = Backbone.Router.extend({
routes: {
"application/index/journal/:id": "journal",
"application/index/account/:id": "account",
"application/index/activity/:id": "activity",
"application/index/register": "register",
"application/index/index": "index",
"application/index/*": "index",
"application/*": "index",
"": "index",
"*actions": "defaultRoute" // Backbone will try match the route above first
}
});
// Initiate the router
var app_router = new AppRouter;
app_router.on('route:journal', function (id) {
//instantiate a controller
// alert("Journal");
});
app_router.on('route:account', function(id) {
//instantiate a controller
// alert("Account");
});
app_router.on('route:activity', function (id) {
//instantiate a controller
// alert("Activity");
});
app_router.on('route:register', function() {
new App.views.register();
});
app_router.on('route:index', function () {
//instantiate a controller
// alert("Index");
});
app_router.on('route:defaultRoute', function() {
//instantiate a controller
// alert("Default Route");
});
// Start Backbone history a necessary step for bookmarkable URL's
Backbone.history.start({pushState: true});
<file_sep><?php
namespace DoctrineMongoODMModule\Hydrator;
use Doctrine\ODM\MongoDB\DocumentManager;
use Doctrine\ODM\MongoDB\Mapping\ClassMetadata;
use Doctrine\ODM\MongoDB\Hydrator\HydratorInterface;
use Doctrine\ODM\MongoDB\UnitOfWork;
/**
* THIS CLASS WAS GENERATED BY THE DOCTRINE ODM. DO NOT EDIT THIS FILE.
*/
class ApplicationModelJournalModelHydrator implements HydratorInterface
{
private $dm;
private $unitOfWork;
private $class;
public function __construct(DocumentManager $dm, UnitOfWork $uow, ClassMetadata $class)
{
$this->dm = $dm;
$this->unitOfWork = $uow;
$this->class = $class;
}
public function hydrate($document, $data, array $hints = array())
{
$hydratedData = array();
/** @Field(type="id") */
if (isset($data['_id'])) {
$value = $data['_id'];
$return = $value instanceof \MongoId ? (string) $value : $value;
$this->class->reflFields['id']->setValue($document, $return);
$hydratedData['id'] = $return;
}
/** @ReferenceOne */
if (isset($data['user'])) {
$reference = $data['user'];
if (isset($this->class->fieldMappings['user']['simple']) && $this->class->fieldMappings['user']['simple']) {
$className = $this->class->fieldMappings['user']['targetDocument'];
$mongoId = $reference;
} else {
$className = $this->dm->getClassNameFromDiscriminatorValue($this->class->fieldMappings['user'], $reference);
$mongoId = $reference['$id'];
}
$targetMetadata = $this->dm->getClassMetadata($className);
$id = $targetMetadata->getPHPIdentifierValue($mongoId);
$return = $this->dm->getReference($className, $id);
$this->class->reflFields['user']->setValue($document, $return);
$hydratedData['user'] = $return;
}
/** @Field(type="string") */
if (isset($data['type'])) {
$value = $data['type'];
$return = (string) $value;
$this->class->reflFields['type']->setValue($document, $return);
$hydratedData['type'] = $return;
}
/** @Field(type="date") */
if (isset($data['timestamp'])) {
$value = $data['timestamp'];
if ($value instanceof \MongoDate) { $return = new \DateTime(); $return->setTimestamp($value->sec); } elseif (is_numeric($value)) { $return = new \DateTime(); $return->setTimestamp($value); } else { $return = new \DateTime($value); }
$this->class->reflFields['timestamp']->setValue($document, clone $return);
$hydratedData['timestamp'] = $return;
}
/** @Field(type="string") */
if (isset($data['entry'])) {
$value = $data['entry'];
$return = (string) $value;
$this->class->reflFields['entry']->setValue($document, $return);
$hydratedData['entry'] = $return;
}
/** @Field(type="boolean") */
if (isset($data['fromSMS'])) {
$value = $data['fromSMS'];
$return = (bool) $value;
$this->class->reflFields['fromSMS']->setValue($document, $return);
$hydratedData['fromSMS'] = $return;
}
/** @Field(type="boolean") */
if (isset($data['fromSystem'])) {
$value = $data['fromSystem'];
$return = (bool) $value;
$this->class->reflFields['fromSystem']->setValue($document, $return);
$hydratedData['fromSystem'] = $return;
}
/** @Field(type="date") */
if (isset($data['createdOn'])) {
$value = $data['createdOn'];
if ($value instanceof \MongoDate) { $return = new \DateTime(); $return->setTimestamp($value->sec); } elseif (is_numeric($value)) { $return = new \DateTime(); $return->setTimestamp($value); } else { $return = new \DateTime($value); }
$this->class->reflFields['createdOn']->setValue($document, clone $return);
$hydratedData['createdOn'] = $return;
}
/** @Field(type="date") */
if (isset($data['modifiedOn'])) {
$value = $data['modifiedOn'];
if ($value instanceof \MongoDate) { $return = new \DateTime(); $return->setTimestamp($value->sec); } elseif (is_numeric($value)) { $return = new \DateTime(); $return->setTimestamp($value); } else { $return = new \DateTime($value); }
$this->class->reflFields['modifiedOn']->setValue($document, clone $return);
$hydratedData['modifiedOn'] = $return;
}
/** @Field(type="string") */
if (isset($data['msisdn'])) {
$value = $data['msisdn'];
$return = (string) $value;
$this->class->reflFields['msisdn']->setValue($document, $return);
$hydratedData['msisdn'] = $return;
}
return $hydratedData;
}
}<file_sep><?php
namespace Application\Controller;
use Zend\View\Model\JsonModel;
use Zend\Json\Json;
use Application\Controller\BaseRestfulController;
use Application\Model\JournalModel;
use Zend\Http\Response;
use Zend\Log\Logger;
use Zend\Log\Writer\Stream;
use Nexmo\NexmoAccount;
use Nexmo\NexmoMessage;
use Nexmo\NexmoReceipt;
class MessageController extends BaseRestfulController
{
private $wakeupMsg = "Hello, our system has detected you recently woke up. How'd you sleep?";
public function receiveMessageAction()
{
$request = $this->getRequest();
$rawdata = $this->getRequest()->getQuery();
$entry = new JournalModel();
$logger = new Logger;
$writer = new Stream('error.log');
$logger->addWriter($writer);
$logger->log(Logger::INFO, 'request uri : '.$request->getUri());
$logger->log(Logger::INFO, 'request method : '.$request->getMethod());
$logger->log(Logger::INFO, 'request query: '.$request->getQuery()->toString());
if($rawdata['msisdn']){
$userRepo = $this->getDocumentManager()->getRepository('Application\Model\UserModel');
$entry->type = "misc";
$entry->entry = $rawdata['text'];
$entry->fromSMS = true;
$entry->fromSystem = false;
$entry->timestamp = strtotime($rawdata['message-timestamp']);
$entry->user = $userRepo->getByNumber($rawdata['msisdn']);
$journalRepo = $this->getDocumentManager()->getRepository('Application\Model\JournalModel');
$newEntry = $journalRepo->create($entry);
$this->getDocumentManager()->flush();
}
$response = new Response();
$response->setStatusCode(Response::STATUS_CODE_200);
$this->response = $response;
return $this->response;
}
public function sendMessageAction()
{
$request = $this->getRequest();
$rawdata = $this->getRequest()->getQuery();
$entry = new JournalModel();
$logger = new Logger;
$writer = new Stream('error.log');
$logger->addWriter($writer);
$logger->log(Logger::INFO, 'request uri : '.$request->getUri());
$logger->log(Logger::INFO, 'request method : '.$request->getMethod());
$logger->log(Logger::INFO, 'request query: '.$request->getQuery()->toString());
if($rawdata['to_number']){
$sms = new NexmoMessage('c034c6ef', '4cde3e0f');
$sms->sendText( $rawdata['to_number'], '17142940264', $this->wakeupMsg );
$userRepo = $this->getDocumentManager()->getRepository('Application\Model\UserModel');
$entry->type = "misc";
$entry->entry = $this->wakeupMsg;
$entry->fromSMS = false;
$entry->fromSystem = true;
$entry->timestamp = time();
$entry->user = $userRepo->getByNumber($rawdata['to_number']);
$journalRepo = $this->getDocumentManager()->getRepository('Application\Model\JournalModel');
$newEntry = $journalRepo->create($entry);
$this->getDocumentManager()->flush();
}
$response = new Response();
$response->setStatusCode(Response::STATUS_CODE_200);
$this->response = $response;
return $this->response;
}
}
<file_sep><?php
namespace Application\Controller;
use Zend\Mvc\Controller\AbstractActionController;
use Zend\View\Model\ViewModel;
use Nexmo\NexmoAccount;
use Nexmo\NexmoMessage;
use Nexmo\NexmoReceipt;
use Application\Model\UserModel;
use Application\Model\JournalModel;
class CronController extends AbstractActionController
{
/**
* @var Doctrine\ODM\MongoDB\DocumentManager
*/
protected $dm;
/**
* @var Application\Mapper\Manager
*/
protected $mManager;
public function getDocumentManager() {
if (null === $this->dm) {
$this->dm = $this->getServiceLocator()->get('doctrine.documentmanager.odm_default');
}
return $this->dm;
}
protected $sleepMsg = "Hey, how'd you sleep?";
protected $foodMsg = "Have you eaten anything today?";
protected $moodMsg = "How has your day been?";
protected $miscMsg = "Is there anything you'd like to add to your journal?";
public function sendMessagesAction()
{
$request = $this->getRequest();
if(null !== $request->getParam('sleep')){
return $this->sendMessageBatch($this->sleepMsg);
}else if(null !== ($request->getParam('food'))){
return $this->sendMessageBatch($this->foodMsg);
}else if(null !== ($request->getParam('mood'))){
return $this->sendMessageBatch($this->moodMsg);
}else if(null !== ($request->getParam('misc'))){
return $this->sendMessageBatch($this->miscMsg);
}
return "sendMessages was called";
}
public function sendMessageBatch($message){
$userRepo = $this->getDocumentManager()->getRepository('Application\Model\UserModel');
$activeUsers = $userRepo->getActiveUsers();
foreach($activeUsers as $user){
$sms = new NexmoMessage('c034c6ef', '4cde3e0f');
$sms->sendText( $user->phone_number, '17142940264', $message );
$userRepo = $this->getDocumentManager()->getRepository('Application\Model\UserModel');
$entry = new JournalModel();
$entry->type = "misc";
$entry->entry = $message;
$entry->fromSMS = false;
$entry->fromSystem = true;
$entry->timestamp = time();
$entry->user = $user;
$journalRepo = $this->getDocumentManager()->getRepository('Application\Model\JournalModel');
$newEntry = $journalRepo->create($entry);
}
$this->getDocumentManager()->flush();
}
}<file_sep>$(function(){
/**
* Set up our entry model
*/
var Entry = Backbone.Model.extend({
defaults: function(){
return {
type: "misc",
entry: "default journal entry",
date: new Date()
}
}
});
/**
* Set up our entries collection
*/
var EntryList = Backbone.Collection.extend({
model: Entry,
localStorage: new Backbone.LocalStorage("entries-backbone"),
comparator: 'date'
});
/**
* Initialize our entries collection
*/
var Entries = new EntryList;
/**
* Set up our entry view
*/
var EntryView = Backbone.View.extend({
tagName: "li",
template: _.template($('#entry-template').html()),
events: {
"click .edit" : "edit",
"click .delete" : "delete"
},
initialize: function() {
this.listenTo(this.model, 'change', this.render);
this.listenTo(this.model, 'destroy', this.remove);
},
render: function() {
this.$el.html(this.template(this.model.toJSON()));
return this;
},
edit: function() {
//open "editing mode"
},
close: function() {
//close "editing mode"
},
clear: function() {
this.model.destroy();
}
});
/**
* Set up our top level UI element
*/
var AppView = Backbone.View.extend({
el: $("#journalapp"),
events: {
"click #new-entry": "createNewEntry"
},
initialize: function() {
this.listenTo(Entries, 'add', this.addOne);
this.listenTo(Entries, 'reset', this.addAll);
this.listenTo(Entries, 'all', this.render);
this.main = $('#main');
Entries.fetch();
},
});
});
function getCurrentDate(){
var today = new Date();
var dd = today.getDate();
var mm = today.getMonth()+1; //January is 0!
var yyyy = today.getFullYear();
if(dd<10) {
dd='0'+dd
}
if(mm<10) {
mm='0'+mm
}
today = mm+'/'+dd+'/'+yyyy;
return today;
}
<file_sep><?php
namespace Application\Controller;
use Zend\Mvc\Controller\AbstractRestfulController;
use Zend\View\Model\JsonModel;
use Zend\EventManager\EventManagerInterface;
use Zend\Json\Json;
use Application\Controller\BaseRestfulContoller;
use Application\Model\UserModel;
class UserController extends BaseRestfulController
{
public function createAction()
{
$rawdata = json_decode($this->getRequest()->getContent(), true);
$user = new UserModel();
$user->email = $rawdata['email'];
$user->password = $rawdata['password'];
$user->phone_number = $rawdata['phone_number'];
$user->name = $rawdata['name'];
$userRepo = $this->getDocumentManager()->getRepository('Application\Model\UserModel');
$newUser = $userRepo->create($user);
$this->getDocumentManager()->flush();
$this->response->setStatusCode(201);
return new JsonModel(array('message'=>'msg', 'id' => $newUser->id));
}
public function activateAction()
{
$user = $this->getAuthenticatedUser();
$rawdata = json_decode($this->getRequest()->getContent(), true);
$user->status = "active";
$userRepo = $this->getDocumentManager()->getRepository('Application\Model\UserModel');
$newUser = $userRepo->update($user);
$this->getDocumentManager()->flush();
return new JsonModel(array('message'=>'success'));
}
public function changePasswordAction()
{
$user = $this->getAuthenticatedUser();
$rawdata = json_decode($this->getRequest()->getContent(), true);
$userRepo = $this->getDocumentManager()->getRepository('Application\Model\UserModel');
$user->password = $rawdata['<PASSWORD>'];
$user = $userRepo->encrypt($user);
$userRepo->update($user);
$this->getDocumentManager()->flush();
$message = "success";
return new JsonModel(array('message'=>$message));
}
/**
* Delete an existing resource
*
* @param mixed $id
* @return mixed
*/
public function delete($id)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Delete the entire resource collection
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.1.0); instead, raises an exception if not implemented.
*
* @return mixed
*/
public function deleteList()
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Return single resource
*
* @param mixed $id
* @return mixed
*/
public function get($id)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Return list of resources
*
* @return mixed
*/
public function getList()
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Retrieve HEAD metadata for the resource
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.1.0); instead, raises an exception if not implemented.
*
* @param null|mixed $id
* @return mixed
*/
public function head($id = null)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Respond to the PATCH method
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.1.0); instead, raises an exception if not implemented.
*
* @param $id
* @param $data
*/
public function patch($id, $data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Replace an entire resource collection
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.1.0); instead, raises an exception if not implemented.
*
* @param mixed $data
* @return mixed
*/
public function replaceList($data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Modify a resource collection without completely replacing it
*
* Not marked as abstract, as that would introduce a BC break
* (introduced in 2.2.0); instead, raises an exception if not implemented.
*
* @param mixed $data
* @return mixed
*/
public function patchList($data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
/**
* Update an existing resource
*
* @param mixed $id
* @param mixed $data
* @return mixed
*/
public function update($id, $data)
{
$this->response->setStatusCode(405);
return array(
'content' => 'Method Not Allowed'
);
}
}
| bbc383887de3d8a8c181d30f5a5f14b61a3452bb | [
"JavaScript",
"PHP",
"Markdown"
] | 19 | JavaScript | jdk243/SMSjournal | 19a041e71f6932fdf6d8ac8b3b3739427e0c664f | f2cab18db21cbb1a4774a874c6dda78419ed357f | |
refs/heads/master | <file_sep>file(REMOVE_RECURSE
"CMakeFiles/conversion_function.dir/main.cpp.o"
"conversion_function.pdb"
"conversion_function"
)
# Per-language clean rules from dependency scanning.
foreach(lang CXX)
include(CMakeFiles/conversion_function.dir/cmake_clean_${lang}.cmake OPTIONAL)
endforeach()
<file_sep>cmake_minimum_required(VERSION 3.13)
project(Observer)
set(CMAKE_CXX_STANDARD 14)
add_executable(Observer main.cpp Subject.h Subject.cpp Observer.h Observer.cpp Observer1.h Observer1.cpp)<file_sep>cmake_minimum_required(VERSION 3.13)
project(Complex)
set(CMAKE_CXX_STANDARD 14)
add_executable(Complex main.cpp complex.cpp complex.h my_complex.h my_complex.cpp)<file_sep>#ifndef OBSERVER_SUBJECT_H
#define OBSERVER_SUBJECT_H
#include <vector>
#include <iostream>
#include "Observer.h"
using namespace std;
class Subject{
public:
Subject(int val = 0) {
value = val;
observers.reserve(10);
}
void addObserver(Observer& observer) {
observers.push_back(&observer);
}
void setValue(int val) {
value = val;
notify();
}
void notify() {
for(size_t i = 0; i < observers.size(); i++)
observers[i]->update(value);
}
private:
int value;
vector<Observer*> observers;
};
#endif
<file_sep>cmake_minimum_required(VERSION 3.13)
project(template_pattern)
set(CMAKE_CXX_STANDARD 14)
add_executable(template_pattern main.cpp library.h library.cpp application.h application.cpp)<file_sep>#ifndef OBSERVER_OBSERVER1_H
#define OBSERVER_OBSERVER1_H
#include <iostream>
#include "Observer.h"
#include "Subject.h"
using namespace std;
class Subject;
class Observer1:public Observer{
public:
Observer1(int id = 0) {
m_id = id;
}
Observer1(Subject& s, int id = 0) {
s.addObserver(*this);
m_id = id;
}
virtual void update(int val) {
cout << "Observer1id:" << m_id << " value:" << val << endl;
}
private:
int m_id;
};
#endif
<file_sep>#include "my_complex.h"
inline
my_complex& __doaplus(my_complex* ths, const my_complex& c){
ths->re += c.re;
ths->im += c.im;
return *ths;
}
inline
my_complex& my_complex::operator += (const my_complex &c){
return __doaplus(this, c);
}
ostream& operator << (ostream& os, const my_complex c) {
os << c.real() << "+" << c.image() << "i" << endl;
return os;
}<file_sep>cmake_minimum_required(VERSION 3.14)
project(conversion_function)
set(CMAKE_CXX_STANDARD 14)
add_executable(conversion_function main.cpp Fraction.h)<file_sep>#include "complex.h"
// 类定义
inline complex& complex::operator+=(const complex & r) {
// 所有的成员函数都包含一个隐藏的this函数
return __doap1(this, r);
}
// 第一个传入参数被修改了,所以不用const修饰,第二个参数不进行修改,利用const修饰,同时pass-by-reference
// 返回值为引用类型,因为返回的对象的空间本来就存在,所以可以pass-by-reference
// 如果是实现+而不是+=,则会在函数内部创建新的临时对象,此时必须pass-by-value
inline complex& __doap1(complex* ths, const complex &r)
{
// 友元函数中可以自由取得friend的私有成员
// 注意:相同类的各个object互为友元
ths->re += r.re;
ths->im += r.im;
return *ths;
}
// 此处返回不可以pass-by-reference
complex operator + (const complex& x, const complex& y)
{
// 临时变量
return complex(x.real() + y.real(), x.image() + y.real());
}<file_sep>#include <iostream>
#include "operator_new_delete.h"
int main() {
cout << "----------------array new_delete----------" << endl;
my_operator* m_new_delete = new my_operator[3];
delete[] m_new_delete;
cout << "----------------new_delete---------------" << endl;
m_new_delete = new my_operator(2, 6);
delete m_new_delete;
return 0;
}<file_sep>cmake_minimum_required(VERSION 3.14)
project(operator_new)
set(CMAKE_CXX_STANDARD 14)
add_executable(operator_new main.cpp operator_new_delete.cpp operator_new_delete.h)<file_sep>
#ifndef CONVERSION_FUNCTION_FRACTION_H
#define CONVERSION_FUNCTION_FRACTION_H
class Fraction {
public:
// explicit防止其他类隐式转换成本类
explicit Fraction(int n, int d = 1)
: m_numerator(n), m_denominator(d) { }
// conversion function转换函数,将本类转换成其他类
operator double() const {
return (double)m_numerator/m_denominator;
}
Fraction operator + (const Fraction&f) {
return Fraction(f.m_numerator * m_denominator + f.m_denominator * m_numerator,
f.m_denominator * m_denominator);
}
private:
int m_numerator;
int m_denominator;
};
#endif //CONVERSION_FUNCTION_FRACTION_H
<file_sep>//
// Created by bisheng on 19-4-23.
//
#include "library.h"
void Library::run()
{
Step1();
Step2();
Step3();
Step4();
Step5();
}
void Library::Step1()
{
std::cout << "Step1" << std::endl;
}
void Library::Step3()
{
std::cout << "Step3" << std::endl;
}
void Library::Step5()
{
std::cout << "Step5" << std::endl;
}
<file_sep>
#ifndef SINGLETON_SINGLETON_H
#define SINGLETON_SINGLETON_H
#include <iostream>
class Singleton;
class Singleton
{
public:
static Singleton& getInstance();//static 关键字
void function() {//function公有成员
std::cout << "function" << std::endl;
}
private:
Singleton() { }//构造函数私有成员
};
#endif //SINGLETON_SINGLETON_H
<file_sep># The set of languages for which implicit dependencies are needed:
set(CMAKE_DEPENDS_LANGUAGES
"CXX"
)
# The set of files for implicit dependencies of each language:
set(CMAKE_DEPENDS_CHECK_CXX
"C:/Users/BiShe/Desktop/cpp/C++OOP/Design pattern/template_pattern/application.cpp" "C:/Users/BiShe/Desktop/cpp/C++OOP/Design pattern/template_pattern/cmake-build-debug/CMakeFiles/template_pattern.dir/application.cpp.obj"
"C:/Users/BiShe/Desktop/cpp/C++OOP/Design pattern/template_pattern/library.cpp" "C:/Users/BiShe/Desktop/cpp/C++OOP/Design pattern/template_pattern/cmake-build-debug/CMakeFiles/template_pattern.dir/library.cpp.obj"
"C:/Users/BiShe/Desktop/cpp/C++OOP/Design pattern/template_pattern/main.cpp" "C:/Users/BiShe/Desktop/cpp/C++OOP/Design pattern/template_pattern/cmake-build-debug/CMakeFiles/template_pattern.dir/main.cpp.obj"
)
set(CMAKE_CXX_COMPILER_ID "GNU")
# The include file search paths:
set(CMAKE_CXX_TARGET_INCLUDE_PATH
)
# Targets to which this target links.
set(CMAKE_TARGET_LINKED_INFO_FILES
)
# Fortran module output directory.
set(CMAKE_Fortran_TARGET_MODULE_DIR "")
<file_sep>#include <iostream>
#include "var_template.h"
int main() {
print(1, "hello", "world", 1);
return 0;
}<file_sep>#include <iostream>
#include "mystring.h"
#include "String.h"
using namespace std;
int main() {
return 0;
}<file_sep>#include <iostream>
#include "plus.h"
using namespace std;
int main() {
myplus<int> p;
auto result1 = p(5, 3);
auto result2 = myplus<int>() (2, 6);
cout << result1 << endl;
cout << result2 << endl;
return 0;
}<file_sep>//
// Created by bisheng on 19-4-23.
//
#ifndef TEMPLATE_PATTERN_LIBRARY_H
#define TEMPLATE_PATTERN_LIBRARY_H
#include <iostream>
class Library
{
public:
Library(){}
// 模板,即算法骨架(稳定)
void run();
virtual ~Library(){};
private:
void Step1();
void Step3();
void Step5();
protected:
// 算法的某些步骤 (变化),延迟到子类中实现
virtual void Step2() {};
virtual void Step4() {};
};
#endif //TEMPLATE_PATTERN_LIBRARY_H
<file_sep>#ifndef __MYSTRING_H
#include <cstring>
#include <iostream>
using namespace std;
class String;
ostream& operator << (ostream& os, const String& str);
// 此类的特殊之处是成员含有指针
class String
{
public:
// 含有指针的类必须自己定义拷贝构造,拷贝赋值,和析构函数 Big Three
String(const char* cstr = 0);
String(const String& str);
String& operator=(const String& str);
~String();
char* get_c_str() const { return m_data; }
private:
char* m_data;
};
#define __MYSTRING_H
#endif
<file_sep>file(REMOVE_RECURSE
"CMakeFiles/function_like_class.dir/main.cpp.o"
"CMakeFiles/function_like_class.dir/plus.cpp.o"
"function_like_class.pdb"
"function_like_class"
)
# Per-language clean rules from dependency scanning.
foreach(lang CXX)
include(CMakeFiles/function_like_class.dir/cmake_clean_${lang}.cmake OPTIONAL)
endforeach()
<file_sep>#include <iostream>
#include "library.h"
#include "application.h"
int main() {
Application *a = new Application();
// 子类可以调用父类函数
a->run();
delete a;
return 0;
}<file_sep>#include "Observer1.h"
<file_sep>cmake_minimum_required(VERSION 3.14)
project(variadic_templates)
set(CMAKE_CXX_STANDARD 14)
add_executable(variadic_templates main.cpp var_template.cpp var_template.h)<file_sep>#ifndef __MY_COMPLEX_H
#define __MY_COMPLEX_H
#include <iostream>
using namespace std;
class my_complex;
ostream& operator << (ostream& os, const my_complex c);
class my_complex {
public:
my_complex(double r = 0, double i = 0): re(r), im(i)
{ }
double real() const{ return re; }
double image() const{ return im; }
my_complex& operator += (const my_complex&);
private:
double re, im;
friend my_complex& __doaplus(my_complex*, const my_complex&);
};
#endif<file_sep>#include "var_template.h"
void print(void)
{
return;
}<file_sep>#include <iostream>
#include "my_complex.h"
#include "complex.h"
using namespace std;
int main() {
my_complex c(1, 2);
cout << c << endl;
return 0;
}<file_sep>#include "Singleton2.h"
Singleton2 Singleton2::s;
Singleton2& Singleton2::getInstance() {
return s;
}<file_sep>#ifndef FUNCTION_LIKE_CLASS_PLUS_H
#define FUNCTION_LIKE_CLASS_PLUS_H
template <class T>
class myplus {
public:
T operator()(const T&x, const T&y) const{return x + y;}
};
#endif //FUNCTION_LIKE_CLASS_PLUS_H
<file_sep>#ifndef SINGLETON_SINGLETON2_H
#define SINGLETON_SINGLETON2_H
#include <iostream>
using namespace std;
class Singleton2;
class Singleton2
{
public:
static Singleton2& getInstance();
void function() { cout << "function has been called" << endl;}
private:
Singleton2() { }
static Singleton2 s;
};
#endif //SINGLETON_SINGLETON2_H
<file_sep>#ifndef POINTER_LIKE_CLASS_SHARE_PTR_H
#define POINTER_LIKE_CLASS_SHARE_PTR_H
template <class T>
class share_ptr {
public:
share_ptr() { }
share_ptr(T* p): ptr(p) { }
T* operator = (T* p) {
ptr = p;
return ptr;
}
T operator*() {
return *ptr;
}
T* operator->() {
return ptr;
}
private:
T* ptr;
long* value;
};
#endif
<file_sep>#include <iostream>
#include <vector>
#include "Subject.h"
#include "Observer.h"
#include "Observer1.h"
using namespace std;
int main() {
Subject s;
Observer1 o1;
Observer1 o2(s, 1);
s.addObserver(o1);
s.setValue(5);
return 0;
}<file_sep>#include "mystring.h"
mystring::mystring(const char *cstr) {
if(!cstr) {
m_data = new char[1];
m_data[0] = '\0';
}
else {
m_data = new char[strlen(cstr) + 1];
strcpy(m_data, cstr);
}
}
mystring::mystring(const mystring& str) {
size_t length = strlen(str.m_data);
m_data = new char[length + 1];
strcpy(m_data, str.m_data);
}
mystring& mystring::operator=(const mystring& str) {
if(this == &str)
return *this;
delete[] m_data;
m_data = new char[strlen(str.m_data) + 1];
strcpy(m_data, str.m_data);
return *this;
}
mystring::~mystring() {
delete[] m_data;
}
ostream& operator << (ostream& os, const mystring& str){
os << str.get_cstr();
return os;
}<file_sep>#include "Singleton.h"
// static必须在.cpp成员中定义,不能在.h文件中定义
Singleton& Singleton::getInstance() {
static Singleton s;//static关键字
return s;
}<file_sep>//
// Created by bisheng on 19-4-23.
//
#ifndef TEMPLATE_PATTERN_APPLICATION_H
#define TEMPLATE_PATTERN_APPLICATION_H
#include "library.h"
#include <iostream>
class Application:public Library
{
public:
Application(){}
~Application(){}
virtual void Step2();
virtual void Step4();
};
#endif //TEMPLATE_PATTERN_APPLICATION_H
<file_sep>cmake_minimum_required(VERSION 3.13)
project(String)
set(CMAKE_CXX_STANDARD 14)
add_executable(String main.cpp mystring.h mystring.cpp String.h String.cpp)<file_sep>#include <iostream>
#include "share_ptr.h"
using namespace std;
int main() {
share_ptr<int> p;
int* p1 = new int(6);
p = p1;
cout << *p << endl;
return 0;
}<file_sep>#include "share_ptr.h"
<file_sep>#ifndef OPERATOR_NEW_OPERATOR_NEW_H
#define OPERATOR_NEW_OPERATOR_NEW_H
#include <cstdlib>
#include <iostream>
using namespace std;
class my_operator {
public:
my_operator(double r = 0, double i = 0): re(r), im(i) { cout << "ctor" << endl;}
~my_operator(){ cout << "dtor" << endl; }
void* operator new (size_t size){
void* p = malloc(size);
cout << "my operator new, size:" << size << endl;
return p;
}
void* operator new[] (size_t size){
void* p = malloc(size);
cout << "my operator new, size:" << size << endl;
return p;
}
void operator delete(void * p) {
free(p);
cout << "my operator delete" << endl;
}
void operator delete[](void * p) {
free(p);
cout << "my operator delete" << endl;
}
private:
double re, im;
};
#endif //OPERATOR_NEW_OPERATOR_NEW_H
<file_sep>file(REMOVE_RECURSE
"CMakeFiles/template_pattern.dir/main.cpp.obj"
"CMakeFiles/template_pattern.dir/library.cpp.obj"
"CMakeFiles/template_pattern.dir/application.cpp.obj"
"template_pattern.pdb"
"template_pattern.exe"
"template_pattern.exe.manifest"
"libtemplate_pattern.dll.a"
)
# Per-language clean rules from dependency scanning.
foreach(lang CXX)
include(CMakeFiles/template_pattern.dir/cmake_clean_${lang}.cmake OPTIONAL)
endforeach()
<file_sep>cmake_minimum_required(VERSION 3.13)
project(Singleton)
set(CMAKE_CXX_STANDARD 14)
add_executable(Singleton main.cpp Singleton.h Singleton.cpp Singleton2.h Singleton2.cpp)<file_sep>//
// Created by bisheng on 19-4-23.
//
#include "application.h"
void Application::Step2()
{
std::cout << "Step2" << std::endl;
}
void Application::Step4()
{
std::cout << "Step4" << std::endl;
}
<file_sep>#include <iostream>
#include "Singleton.h"
#include "Singleton2.h"
int main() {
Singleton2::getInstance().function();
return 0;
}<file_sep>#include "operator_new_delete.h"
<file_sep>#ifndef VARIADIC_TEMPLATES_VAR_TEMPLATE_H
#define VARIADIC_TEMPLATES_VAR_TEMPLATE_H
#include <iostream>
using namespace std;
void print(void);
template<typename T, typename... Types>
void print(const T& firstArg, const Types&... args)
{
cout << firstArg << endl;
if(sizeof...(args) > 0)
print(args...);
else return;
}
#endif //VARIADIC_TEMPLATES_VAR_TEMPLATE_H
<file_sep>cmake_minimum_required(VERSION 3.14)
project(function_like_class)
set(CMAKE_CXX_STANDARD 14)
add_executable(function_like_class main.cpp plus.cpp plus.h)<file_sep>// 防卫式声明
#ifndef __COMPLEX
#define __COMPLEX
// 前置声明
class complex;
complex operator + (const complex& x, const complex& y);
// 类声明
class complex{
public:
// 构造函数初始化列表
complex(double r = 0, double i = 0) : re(r), im(i)
{ }
// 参数传递尽量使用reference,但是为了防止函数内部对传入参数的修改,利用const关键字进行修饰
// 返回值也尽量使用reference,返回值什么时候可以使用reference,什么时候不可以
complex& operator += (const complex&);
// const函数
double real() const { return re;}
double image() const { return im;}
private:
// 数据放在private中
double re, im;
// 友元函数
friend complex& __doap1(complex* ths, const complex& c);
};
#endif
<file_sep>#ifndef OBSERVER_OBSERVER_H
#define OBSERVER_OBSERVER_H
class Observer {
public:
virtual void update(int val) = 0;
virtual ~Observer() {}
};
#endif
<file_sep>#ifndef __MY_STRING_H
#define __MY_STRING_H
#include <cstring>
#include <iostream>
using namespace std;
class mystring;
ostream& operator << (ostream& os, const mystring& str);
class mystring {
public:
mystring(const char* cstr = 0);
mystring(const mystring& str);
mystring& operator = (const mystring& str);
~mystring();
char* get_cstr() const
{ return m_data;}
private:
char * m_data;
};
#endif<file_sep>#include <iostream>
#include "Fraction.h"
int main() {
Fraction f(1, 2);
double d = f + 4.;
std::cout << d << std::endl;
return 0;
}<file_sep>file(REMOVE_RECURSE
"CMakeFiles/pointer_like_class.dir/main.cpp.o"
"CMakeFiles/pointer_like_class.dir/share_ptr.cpp.o"
"pointer_like_class.pdb"
"pointer_like_class"
)
# Per-language clean rules from dependency scanning.
foreach(lang CXX)
include(CMakeFiles/pointer_like_class.dir/cmake_clean_${lang}.cmake OPTIONAL)
endforeach()
<file_sep>cmake_minimum_required(VERSION 3.14)
project(pointer_like_class)
set(CMAKE_CXX_STANDARD 14)
add_executable(pointer_like_class main.cpp share_ptr.cpp share_ptr.h) | bb3689353c6cbb33968e3bc634d77dad104fe50f | [
"CMake",
"C++"
] | 52 | CMake | BishengSJTU/Cpp-Houjie | 46cf1e1af201d621c4c24f6a5bae53e629edc97f | d579100d5b1b50fcd58aa8bb5fa926b58b7bb54b | |
refs/heads/master | <repo_name>TTFlyzebra/phpxinyi<file_sep>/Apps/Admin/Controller/AjaxController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
class AjaxController extends Controller {
public function index(){
echo __ACTION__;
}
public function log(){
if (!IS_POST) $this->error("页面不存在!");
$data = $_POST;
if ($data['shopname'] == "") die("no shopname!");
$user = M ( 'shop' )->where(array('shopname'=>$data['shopname']))->find();
if(!$user) die ( '用户未注册!' );
if ($data['loginword'] == "") die("no loginword!");
if ($user['loginword']!=md5($data['loginword'])) die("密码错误!");
}
public function reg(){
if (!IS_POST) $this->error("页面不存在!");
$data = $_POST;
if ($data['shopname'] == "") die("no shopname!");
$count = M ( 'shop' )->where(array('shopname'=>$data['shopname']))->find();
if ($count) die ( '商店名称已注册,请尝试在商店名称前加上地址信息以区分!' );
}
}<file_sep>/Apps/Admin/Public/js/admin-product.js
$(document).ready(function() {
$('#addproduct').click(function() {
if ($('#ptype_name').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#ptype_name').focus();
return false;
}
// if ($("input[name='pattr_id[]']:checked").length == 0) {
// $('#checkbox_css').focus().css({
// boxShadow : "0 0 3px #0000ef"
//
// });
// return false;
// } else {
// $('#checkbox_css').focus().css({
// boxShadow : "0 0 0px #0000ef"
// });
// }
if ($('#name').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#name').focus();
return false;
}
if ($('#price').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#price').focus();
return false;
}
//检查输入是否为数字
if (isNaN($('#price').val())) {
$('#price').focus();
return false;
}
//检查输入的小数不能超过2位
if ($('#price').val().split(".").length > 1
&& $('#price').val().split(".")[1].length > 2) {
$('#price').focus();
return false
}
if ($('#proimg1').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#proimg1').focus();
return false;
}
// if ($('#proimg2').val() == "") {
// // alert("请选择分类!");
// // document.getElementById("message").innerHTML = "名称不能为空!";
// $('#proimg2').focus();
// return false;
// }
// if ($('#proimg3').val() == "") {
// // alert("请选择分类!");
// // document.getElementById("message").innerHTML = "名称不能为空!";
// $('#proimg3').focus();
// return false;
// }
if ($('#describe').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#describe').focus();
return false;
}
$('#addFrom').submit();
});
});
<file_sep>/Apps/Admin/Controller/NodeController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
class NodeController extends AuthController {
/**
* 显示节点管理页面
*/
public function index() {
$node = M ( 'node' )->select ();
// $showArray = array();
// foreach($node as $temp){
// }
// $this->showdata = $node;
$node=node_merge($node);
$this->node=$node;
$this->display ();
}
/**
* 添加节点
*/
public function add() {
if (! IS_POST)$this->error ( "页面不存在!" );
if ($_POST ['node_name'] == null
|| $_POST ['node_status'] == null
|| $_POST ['node_remark'] == null
|| $_POST ['node_pid'] == null
|| $_POST ['node_level'] == null) {
$this->error ( "传入参数错误!" );
}
$node = array (
'name' => $_POST ['node_name'],
'status' => $_POST ['node_status'],
'remark' => $_POST ['node_remark'],
'pid' => $_POST ['node_pid'],
'level' => $_POST ['node_level']
);
if (M ( 'node' )->add ( $node )) {
$this->redirect ( "/Admin/node" );
} else {
$this->error ( "添加节点错误!" );
}
}
/**
* 修改节点
*/
public function edit() {
if (! IS_POST)$this->error ( "页面不存在!" );
if ($_POST ['node_id'] == null
||$_POST ['node_name'] == null
|| $_POST ['node_status'] == null
|| $_POST ['node_remark'] == null
|| $_POST ['node_pid'] == null
|| $_POST ['node_level'] == null) {
$this->error ( "传入参数错误!" );
}
$node = array (
'id'=>$_POST ['node_id'],
'name' => $_POST ['node_name'],
'status' => $_POST ['node_status'],
'remark' => $_POST ['node_remark'],
'pid' => $_POST ['node_pid'],
'level' => $_POST ['node_level']
);
if (M ( 'node' )->save ( $node )) {
$this->redirect ( "/Admin/node" );
} else {
$this->error ( "修改结点失败!" );
}
}
/**
* 删除节点
*/
public function del() {
if (! IS_POST) $this->error ( "页面不存在!" );
$node = array (
'id' => $_POST ['id'],
'name' => $_POST ['name'],
'status' => $_POST ['status'],
'remark' => $_POST ['remark'],
);
M ( 'node' )->where (array($node ))->delete ();
}
/**
* 修改节点状态
*/
public function status() {
if (! IS_POST) $this->error ( "页面不存在!" );
$node = array (
'id' => $_POST ['id'],
'name' => $_POST ['name'],
'status' => $_POST ['status'],
'remark' => $_POST ['remark'],
);
M ( 'node' )->where($node)->save(array('status'=>!$node['status']));
}
}<file_sep>/Apps/Home/Controller/WeixinPayController.class.php
<?php
namespace Home\Controller;
use Think\Controller;
class WeixinPayController extends Controller {
public function index() {
header('Content-Type: text/xml');
$postData = "<xml>
<appid>wxb4ba3c02aa476ea1</appid>
<attach>支付测试</attach>
<body>APP支付测试</body>
<mch_id>1305176001</mch_id>
<nonce_str>1add1a30ac87aa2db72f57a2375d8fec</nonce_str>
<notify_url>http://wxpay.weixin.qq.com/pub_v2/pay/notify.v2.php</notify_url>
<out_trade_no>1415659990</out_trade_no>
<spbill_create_ip>192.168.3.11</spbill_create_ip>
<total_fee>1</total_fee>
<trade_type>APP</trade_type>
<sign>ce187ed67e05c2d8879bf66bbfdfc8b9</sign>
</xml> ";
$data = vpost ("https://api.mch.weixin.qq.com/pay/unifiedorder", $postData);
// $data = vpost ("https://flyzebra.wicp.net/xinyi/API/Product", "");
echo $data;
}
}<file_sep>/Apps/Common/Model/ShopRoleModel.class.php
<?php
namespace Common\Model;
use Think\Model\RelationModel;
class ShopRoleModel extends RelationModel{
//定义主表
protected $tableName = 'shop';
//定义关联关系
protected $_link = array(
'role'=>array(
'mapping_type' => self::MANY_TO_MANY,
'class_name' => 'role',
'foreign_key'=>'user_id',
'relation_key'=>'role_id',
'relation_table'=>'fz_role_user',
'mapping_fields'=>'id,remark'
)
);
}<file_sep>/Runtime/Cache/Admin/831ccbd780db7bbeb6d94534274f2132.php
<?php if (!defined('THINK_PATH')) exit();?><!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>宇翔科技后台管理</title>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/jquery-1.9.0.min.js"></script>
<script type="text/javascript">
var ajaxadd = '<?php echo U("/Admin/HomeShop/add");?>'
var ajaxdel = '<?php echo U("/Admin/HomeShop/del");?>'
</script>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/admin-homeshop.js"></script>
</head>
<body>
<div class="crumb-wrap">
<span class="icon-font">  <a href="<?php echo U('/Admin');?>">首页</a></span> <span class="crumb-step">> <a href="#">首页推荐商店设置</a></span>
</div>
<div class="main-wrap">
<div class="result-wrap">
<table class="admin-tab" width="100%">
<tbody align="right">
<tr style="background:#00e8e8">
<th colspan="7">首页展示商店</th>
</tr>
<tr style="background:#e8e8e8">
<th width="14%">商店名称</th>
<th width="8%">店长姓名</th>
<th width="10%">店长电话</th>
<th width="10%">商店电话</th>
<th width="13%">注册时间</th>
<th >商店地址</th>
<th width="12%">执行操作</th>
</tr>
<?php if(is_array($shops)): foreach($shops as $key=>$v): if($v['show'] == 1): ?><tr>
<td><?php echo ($v["shopname"]); ?></td>
<th><?php echo ($v["peoplename"]); ?></th>
<th><?php echo ($v["peoplephone"]); ?></th>
<th><?php echo ($v["shopphone"]); ?></th>
<th><?php echo (date("Y-m-d H:i",$v["regtime"])); ?></th>
<td><?php echo ($v["address"]); ?></td>
<th><a href="javascript:delShow('<?php echo ($v["id"]); ?>');"><i class="btn btn-primary">取消展示</i></a></th>
</tr><?php endif; endforeach; endif; ?>
</tbody>
</table>
<br>
<!--input class="common-text" placeholder="商店名称" name="keywords" size="40" value="" id="" type="text">
<input class="btn btn-primary btn2" name="sub" value="搜索商店" type="submit"-->
<table class="admin-tab" width="100%">
<tbody align="right">
<tr style="background:#00e8e8">
<th colspan="7">首页未展示商店</th>
</tr>
<tr style="background:#e8e8e8">
<th width="14%">商店名称</th>
<th width="8%">店长姓名</th>
<th width="10%">店长电话</th>
<th width="10%">商店电话</th>
<th width="13%">注册时间</th>
<th >商店地址</th>
<th width="12%">执行操作</th>
</tr>
<?php if(is_array($shops)): foreach($shops as $key=>$v): if($v['show'] != 1): ?><tr>
<td><?php echo ($v["shopname"]); ?></td>
<th><?php echo ($v["peoplename"]); ?></th>
<th><?php echo ($v["peoplephone"]); ?></th>
<th><?php echo ($v["shopphone"]); ?></th>
<th><?php echo (date("Y-m-d H:i",$v["regtime"])); ?></th>
<td><?php echo ($v["address"]); ?></td>
<th><a href="javascript:addShow('<?php echo ($v["id"]); ?>');"><i class="btn btn-primary">首页展示</i></a></th>
</tr><?php endif; endforeach; endif; ?>
</tbody>
</table>
</div>
</div>
<div class="dlg-mask"></div>
<!-- 添加菜单 Start-->
<div class="dlg-content">正在向服务器提交请求.....</div>
</body>
</html><file_sep>/Apps/Admin/Controller/AccessController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
/**
* 配置角色节点
* @author FlyZebra
*
*/
class AccessController extends AuthController {
/**
* 配置角色页面
*/
public function index() {
$r_id = I('pid');
$this->r_id = $r_id;
$access = M('access')->where(array('role_id'=>$r_id))->getField('node_id',true);
$node = M ( 'node' )->select ();
$node = node_merge($node,$access);
$this->node = $node;
$this->role = M ( 'role' )->select ();
$this->access = M ( 'access' )->select ();
$this->display ();
}
/**
* 配置角色节点
*/
public function setAccess(){
$role_id = $_POST['pid'];
$data = array();
foreach ($_POST['nodeid'] as $v){
$tmp = explode('_', $v);
$data[] = array(
'role_id'=>$role_id,
'node_id'=>$tmp[0],
'level'=>$tmp[1]
);
}
$db= M('access');
$db->where(array('role_id'=>$role_id))->delete();
if($data==null){
$this->redirect("/Admin/Access/index",array('pid' =>$role_id));
}else if($db->addAll($data)){
$this->redirect("/Admin/Access/index",array('pid' =>$role_id));
}else{
$this->error($db->getError());
}
}
}<file_sep>/Runtime/Cache/Admin/0804f70153bdcb52986c9e16a1d6ea93.php
<?php if (!defined('THINK_PATH')) exit();?><!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>宇翔科技后台管理</title>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/jquery-1.9.0.min.js"></script>
<script type="text/javascript"> var ajaxurl= '<?php echo U("/Admin/Attr/del","","");?>'</script>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/admin-attr.js"></script>
</head>
<body>
<div class="crumb-wrap">
<span class="icon-font">  <a href="<?php echo U('/Admin');?>">首页</a></span>
<span class="crumb-step">> <a href="#">商品属性</a></span>
</div>
<div class="main-wrap">
<div class="result-wrap">
<table class="admin-tab" width="900px">
<tbody align="right">
<tr style="background:#e8e8e8">
<th width="30%">属性名称</th>
<th width="30%">加亮颜色</th>
<th width="30%">执行操作</th>
</tr>
<?php if(is_array($pattr)): foreach($pattr as $key=>$v): ?><tr>
<th><?php echo ($v["name"]); ?></th>
<th><div style="margin-left: 135px;vertical-align:middle;width:20px;height:20px;background:<?php echo ($v["color"]); ?>"></div></th>
<th><a href="javascript:postdel('<?php echo ($v["id"]); ?>','<?php echo ($v["name"]); ?>','<?php echo ($v["color"]); ?>');"><i class="icon-font">删除</i></a></th>
</tr><?php endforeach; endif; ?>
</tbody>
</table>
<br>
<form name="addform" id="addform" accept-charset="utf-8" action="<?php echo U('/Admin/Attr/add');?>" method="post">
<table class="admin-tab" width="900px">
<tbody>
<tr>
<th style="background:#e8e8e8" colspan="2">添加属性</th>
</tr>
<tr>
<th width = "200px"><span class="require-red">*</span>属性名称:</th>
<td>
<input class="common-text" id="name" name="name" size="40" value="" type="text">
</td>
</tr>
<tr>
<th><span class="require-red">*</span>属性颜色:</th>
<td>
<input class="common-text" id="color" name="color" size="15" value="#FF0000" type="text"> 以#开头的6位16进制
</td>
</tr>
<tr>
<th></th>
<td>
<input type="submit"class="btn btn-primary btn6 mr10" value="添加" id="add">
<input type="reset" class="btn btn-primary btn6 mr10" value="重置">
</td>
</tr>
</tbody>
</table>
</form>
</div>
</div>
</body>
</html><file_sep>/Apps/Admin/Controller/VerifyController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
/**
* 申核权限
* @author FlyZebra
*
*/
class VerifyController extends AuthController {
/**
* 申核权限页面
*/
public function index() {
$userArr=D('ShopRole')->field('loginword',true)->relation('role')->select();
foreach ( $userArr as $user ) {
$user['role_id'] = $user['role'][0]['id'];
$user['role_remark'] = $user['role'][0]['remark'];
unset($user['role']);
$data[]=$user;
}
$this->shop=$data;
$this->role=M('role')->select();
$this->display ();
}
/**
* 修改用户权限
*/
public function verify(){
$data = $_POST;
$db = M('role_user');
$db->where(array('user_id'=>$data['user_id']))->delete();
if($data['role_id']==null) die("no role_id");
if($db->add($data)){
die("succeed");
}else{
die("failed");
}
}
}<file_sep>/Apps/Common/Conf/config.php
<?php
return array (
// 数据库配置信息
'DB_TYPE' => 'mysql', // 数据库类型
'DB_HOST' => '127.0.0.1', // 服务器地址
'DB_NAME' => 'xinyi', // 数据库名
'DB_USER' => 'root', // 用户名
'DB_PWD' => '<PASSWORD>', // 密码
'DB_PORT' => 3306, // 端口
'DB_PREFIX' => 'fz_', // 数据库表前缀
'DB_CHARSET' => 'utf8', // 字符集
//其它配置
'DEFAULT_MODULE' => 'Home',// 默认模块
'URL_MODEL' => '2', // URL模式
'SESSION_AUTO_START' => true, // 是否开启session
'URL_CASE_INSENSITIVE' => false, //false为默认项,表示URL访问区分大小写
// 'MODULE_ALLOW_LIST' => array('Home','Admin','API'),//允许访问的模块
'URL_HTML_SUFFIX' => '',//修改伪.html后缀
//Cookie设置
// 'COOKIE_EXPIRE' => 0, // Cookie有效期
// 'COOKIE_DOMAIN' => '', // Cookie有效域名
// 'COOKIE_PATH' => '/', // Cookie路径
// 'COOKIE_PREFIX' => '', // Cookie前缀 避免冲突
// 'COOKIE_HTTPONLY' => '', // Cookie的httponly属性 3.2.2新增
// //数据缓存设置
// 'DATA_CACHE_TIME' => 0, // 数据缓存有效期 0表示永久缓存
// 'DATA_CACHE_COMPRESS' => false, // 数据缓存是否压缩缓存
// 'DATA_CACHE_CHECK' => false, // 数据缓存是否校验缓存
// 'DATA_CACHE_PREFIX' => '', // 缓存前缀
// 'DATA_CACHE_TYPE' => 'File', // 数据缓存类型,支持:File|Db|Apc|Memcache|Shmop|Sqlite|Xcache|Apachenote|Eaccelerator
// 'DATA_CACHE_PATH' => TEMP_PATH,// 缓存路径设置 (仅对File方式缓存有效)
// 'DATA_CACHE_SUBDIR' => false, // 使用子目录缓存 (自动根据缓存标识的哈希创建子目录)
// 'DATA_PATH_LEVEL' => 1, // 子目录缓存级别
);
?><file_sep>/Apps/Home/Controller/LoginController.class.php
<?php
namespace Home\Controller;
use Think\Controller;
class LoginController extends Controller {
public function index() {
$this->display ();
}
/**
* 注册用户
*/
public function register() {
if (! IS_POST) $this->error("页面不存在!");
//如果传入的参数没有帐号和密码这两个参数,直接结束。
if($_POST['loginname']==""&&$_POST['loginword']=="") $this->error("传入参数不正确!");
//导入自定义User处理类
import("Cls.User",APP_PATH);
//用户名已存在返回错误信息。
if(\User::verifyName("user", $_POST['loginname'])) die("该用户名已注册!");
//向数据表插入新用户数据。
$data=\User::insertUser("user", $_POST);
if(!$data) $this->error("注册帐号失败,请重试!");
//插入成功,更新用户登陆信息
$log=\User::insertLog("user_log", $data["id"]);
if(!$log) $this->success("注册帐号成功,请登陆!");
//缓存用户信息
\User::saveSession($data, $log);
//跳转到主页
$this->redirect("/");
}
/**
* 验证登陆信息
*/
public function login() {
if (! IS_POST) $this->error("页面不存在!");
//如果传入的参数没有帐号和密码这两个参数,直接结束。
if($_POST['loginname']==""&&$_POST['loginword']=="") $this->error("传入参数不正确!");
//导入自定义User处理类
import("Cls.User",APP_PATH);
$data = \User::verifyUser("user", $_POST['loginname'], $_POST['loginword']);
if($data == -1) $this->error("登陆帐号未注册!");
if($data == 0) $this->error("用户名密码错误!");
//验证通过,更新用度等级//因为用用户登陆次数来判断用户等级
if (!(\User::upUserLevel ('user', $data))) $this->error ("更新数户数据出错,请重试!");
// 更新用户登陆信息
$log = \User::insertLog ( "user_log", $data ["id"] );
if (! $log) $this->success ( "更新用户信息出错,可能服务器连接超时,请重试!" );
// 缓存用户信息
\User::saveSession ( $data, $log );
// 跳转到主页
$this->redirect ( "/" );
}
public function ajax(){
if (!IS_POST) return;
if ($_POST['loginname'] == "") die();
import("Cls.User",APP_PATH);
if ($_POST['loginword'] != "") {
//导入自定义User处理类
$data = \User::verifyUser("user", $_POST['loginname'], $_POST['loginword']);
if ($data==-1) die ( '该用户尚未注册!' );
if ($data==0) die ( '密码输入错误!' );
} else {
if(\User::verifyName("user", $_POST['loginname'])) die("该用户名已注册!");
}
}
}<file_sep>/Apps/Common/Model/ShopAllModel.class.php
<?php
namespace Common\Model;
use Think\Model\RelationModel;
class ShopAllModel extends RelationModel{
//定义主表
protected $tableName = 'shop';
//定义关联关系
protected $_link = array(
'imgurl' => array(
'mapping_type' => self::HAS_ONE,
'class_name' => 'shop_imgurl', //要关联的模型类名
'foreign_key' => 'shop_id', //关联的外键名称
// 'mapping_name' => 'articles',
'mapping_name' => 'imgurl',
// 'mapping_fields'=> 'imageurl',
'as_fields'=>'imgurl',
),
'show'=>array(
'mapping_type' => self::HAS_ONE,
'class_name' => 'shop_home', //要关联的模型类名
'foreign_key' => 'shop_id', //关联的外键名称
'mapping_fields'=> 'show',
'as_fields'=>'show',
)
);
}<file_sep>/Runtime/Cache/Admin/14138ae9c908269cc95601fd8f3b9987.php
<?php if (!defined('THINK_PATH')) exit();?><!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>宇翔科技后台管理</title>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/jquery-1.9.0.min.js"></script>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/md5.js"></script>
<script type="text/javascript"> var ajaxurl= '<?php echo U("/Admin/Ajax/reg");?>'</script>
<link rel="stylesheet" type="text/css" href="/myweb/xinyi/Apps/Admin/Public/css/admin.css" />
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/admin-register.js"></script>
</head>
<body>
<div class="crumb-wrap">
<span class="icon-font">  <a href="<?php echo U('/Admin');?>">首页</a></span> <span class="crumb-step">> <a href="#">注册商店</a></span>
</div>
<div class="main-wrap">
<div class="result-wrap">
<form action="<?php echo U('/Admin/Register/register');?>" method="post" id="registerFrom" name="registerFrom" enctype="multipart/form-data">
<div id="message" class="msg">注册提示信息!</div>
<table class="insert-tab" width="100%">
<tbody>
<tr>
<th width="120"><span class="require-red">*</span>商店名称:</th>
<td><input class="common-text" id="shopname" name="shopname" size="50" value="" type="text"></td>
</tr>
<tr>
<th><span class="require-red">*</span>店主姓名:</th>
<td><input class="common-text" id="peoplename" name="peoplename" size="20" value="" type="text"></td>
</tr>
<tr>
<th><span class="require-red">*</span>密  码:</th>
<td><input class="common-text" id="loginword" name="loginword" size="20" value="" type="password"></td>
</tr>
<tr>
<th><span class="require-red">*</span>确认密码:</th>
<td><input class="common-text" id="loginword2" size="20" value="" type="password"></td>
</tr>
<tr>
<th><span class="require-red">*</span>店主手机:</th>
<td><input class="common-text" id="peoplephone" name="peoplephone" size="20" value="" type="text"></td>
</tr>
<tr>
<th><span class="require-red">*</span>商店电话:</th>
<td><input class="common-text" id="shopphone" name="shopphone" size="20" value="" type="text"></td>
</tr>
<tr>
<th><span class="require-red">*</span>商店地址:</th>
<td><input class="common-text" id="address" name="address" size="100" value="" type="text"></td>
</tr>
<tr>
<th><span class="require-red">*</span>展示图像1:</th>
<td><input class="common-text" name="proimg1" id="proimg1" type="file" accept=".jpg,.png"><span class="require-red">支持格式PNG、JPG,分辨率:480*320。</span></td>
</tr>
<tr>
<th><span class="require-red"> </span>展示图像2:</th>
<td><input class="common-text" name="proimg2" id="proimg2" type="file" accept=".jpg,.png"><span class="require-red">支持格式PNG、JPG,分辨率:480*320。</span></td>
</tr>
<tr>
<th><span class="require-red"> </span>展示图像3:</th>
<td><input class="common-text" name="proimg3" id="proimg3" type="file" accept=".jpg,.png"><span class="require-red">支持格式PNG、JPG,分辨率:480*320。</span></td>
</tr>
<tr>
<th><span class="require-red">*</span>备注信息:</th>
<td><textarea class="common-textarea" name="describe" id="describe" cols="30" style="width: 98%;" rows="10"></textarea></td>
</tr>
<tr>
<th></th>
<td><input type="button" class="btn btn-primary btn6 mr10" value="注册" id="register"> <input type="reset" class="btn btn-primary btn6 mr10" value="重置"></td>
</tr>
</tbody>
</table>
</form>
</div>
</div>
</body>
</html><file_sep>/Runtime/Cache/Admin/ff90e9603868abd64cf17bfaf5ea9be9.php
<?php if (!defined('THINK_PATH')) exit();?>
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>宇翔科技后台管理</title>
<link rel="stylesheet" type="text/css" href="/myweb/xinyi/Apps/Admin/Public/css/node.css" />
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/jquery-1.9.0.min.js"></script>
<script type="text/javascript">
var ajaxurl = '<?php echo U("/Admin/Access");?>'
</script>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/admin-access.js"></script>
</head>
<body>
<div class="crumb-wrap">
<span class="icon-font">  <a href="<?php echo U('/Admin');?>">首页</a></span> <span class="crumb-step">> <a href="#">权限设置</a></span>
</div>
<form name="saveForm" id="saveForm" accept-charset="utf-8" action="<?php echo U('/Admin/Access/setAccess');?>" method="post">
<div class="main-wrap">
<div class="result-wrap">
<div class="apps-title">
<div class="prm-save">
网站目录
<select name="pid" id="pid" class="select-role">
<option value="">请选择角色</option>
<!-- 确定哪一项被选中 -->
<?php if(is_array($role)): foreach($role as $key=>$v): if($v['id'] == $r_id): ?><option value="<?php echo ($v["id"]); ?>" selected="selected"><?php echo ($v["remark"]); ?></option>
<?php else: ?>
<option value="<?php echo ($v["id"]); ?>"><?php echo ($v["remark"]); ?></option><?php endif; endforeach; endif; ?>
</select>
<input type="submit" class="btn btn-primary" value="保存设置" id="save">
</div>
<?php if(is_array($node)): foreach($node as $key=>$v): ?><div class="apps">
<div class="app">
<?php echo ($v["remark"]); ?>
<?php if($v["access"]): ?><input class="checkbox1" type="checkbox" name="nodeid[]" value="<?php echo ($v["id"]); ?>_1" id="1" checked="checked">
<?php else: ?>
<input class="checkbox1" type="checkbox" name="nodeid[]" value="<?php echo ($v["id"]); ?>_1" id="1"><?php endif; ?>
</div>
<?php if(is_array($v["child"])): foreach($v["child"] as $key=>$c): ?><div class="controls">
<div class="control">
<?php echo ($c["remark"]); ?>
<?php if($c["access"]): ?><input class="checkbox1" type="checkbox" name="nodeid[]" value="<?php echo ($c["id"]); ?>_1" id="2" checked="checked">
<?php else: ?>
<input class="checkbox1" type="checkbox" name="nodeid[]" value="<?php echo ($c["id"]); ?>_1" id="2"><?php endif; ?>
</div>
<?php if(is_array($c["child"])): foreach($c["child"] as $key=>$m): ?><div class="method">
<?php echo ($m["remark"]); ?>
<?php if($m["access"]): ?><input class="checkbox1" type="checkbox" name="nodeid[]" value="<?php echo ($m["id"]); ?>_1" id="3" checked="checked">
<?php else: ?>
<input class="checkbox1" type="checkbox" name="nodeid[]" value="<?php echo ($m["id"]); ?>_1" id="3"><?php endif; ?>
</div><?php endforeach; endif; ?>
</div><?php endforeach; endif; ?>
</div><?php endforeach; endif; ?>
</div>
</div>
</div>
</form>
</body>
</html><file_sep>/Apps/Admin/Controller/RegisterController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
class RegisterController extends AuthController {
public function index() {
$this->display ();
// $this->display ( "Register/register" );
}
public function register() {
if (! IS_POST) $this->error ( "页面不存在!" );
// 注册用户
$upload = new \Think\Upload (); // 实例化上传类
$upload->maxSize = 3145728; // 设置附件上传大小
$upload->exts = array ( 'jpg','png'); // 设置附件上传类型
$upload->rootPath = './UpLoads/';
$upload->savePath = '/shop/images/'; // 设置附件上传目录
$data = $_POST;
$data ['regtime'] = time (); // 添加注册时间
$data ['regIP'] = get_client_ip ();
$data ['loginword'] = md5 ( $data ['loginword'] ); // 密码MD5加密
$db = M ( 'shop' );
if ($shop_id = $db->add ( $data )) {
// 接受上传的图像文件
$info = $upload->upload ();
if (! $info) { // 上传错误提示错误信息
$this->error ( "帐号已注册成功,上传图像文件失败,可登陆再修改!" );
} else {
// 更新product_imgurl(产品图像)表
$product_imgurlDao = M ( 'shop_imgurl' );
foreach ( $info as $file ) {
$imgurl = '/UpLoads' . $file ['savepath'] . $file ['savename'];
if (! $product_imgurlDao->add ( array (
'shop_id' => $shop_id,
'imgurl' => $imgurl
) )) {
$this->error ( "帐号已注册成功,添加图像文件失败,可登陆再修改!!" );
} else {
import ( "Fly.MyImage", "." );
\MyImage::thumb ( "." . $imgurl, 480, 320);
}
}
// 处理图像.....图像是上传后处理成统一格式还是显示的时候处理
}
$this->success ( "注册成功!" );
} else {
$this->error ( "注册失败!" );
}
}
}<file_sep>/Apps/Admin/Public/js/admin-attr.js
$(document).ready(function() {
$('#add').click(function() {
if ($('#name').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#name').focus();
return false;
}
if ($('#color').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#color').focus();
return false;
}
$('#addfrom').submit();
});
});
function postdel(param1,param2,param3) {
$.ajax({
url: ajaxurl,
type: 'post',
data: "id="+param1+"&name="+param2+"&color="+param3,
success: function(){
parent.location.reload();
window.location.reload();
},
dataType: 'html'
});
}
<file_sep>/xinyi.sql
-- phpMyAdmin SQL Dump
-- version 4.6.0
-- http://www.phpmyadmin.net
--
-- Host: localhost
-- Generation Time: 2018-03-18 05:35:03
-- 服务器版本: 5.5.42
-- PHP Version: 5.6.20
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- Database: `xinyi`
--
-- --------------------------------------------------------
--
-- 表的结构 `fz_access`
--
CREATE TABLE `fz_access` (
`role_id` smallint(6) UNSIGNED NOT NULL,
`node_id` smallint(6) UNSIGNED NOT NULL,
`level` tinyint(1) NOT NULL,
`module` varchar(50) DEFAULT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_access`
--
INSERT INTO `fz_access` (`role_id`, `node_id`, `level`, `module`) VALUES
(19, 123, 1, NULL),
(19, 122, 1, NULL),
(19, 121, 1, NULL),
(19, 120, 1, NULL),
(19, 119, 1, NULL),
(19, 118, 1, NULL),
(19, 117, 1, NULL),
(19, 116, 1, NULL),
(19, 124, 1, NULL),
(19, 114, 1, NULL),
(19, 113, 1, NULL),
(19, 112, 1, NULL),
(19, 111, 1, NULL),
(19, 110, 1, NULL),
(19, 109, 1, NULL),
(19, 108, 1, NULL),
(19, 107, 1, NULL),
(19, 106, 1, NULL),
(19, 105, 1, NULL),
(19, 104, 1, NULL),
(19, 103, 1, NULL),
(19, 102, 1, NULL),
(19, 101, 1, NULL),
(19, 100, 1, NULL),
(19, 99, 1, NULL),
(19, 98, 1, NULL),
(19, 97, 1, NULL),
(19, 96, 1, NULL),
(19, 95, 1, NULL),
(20, 93, 1, NULL),
(20, 94, 1, NULL),
(20, 95, 1, NULL),
(20, 109, 1, NULL),
(20, 110, 1, NULL),
(20, 111, 1, NULL),
(20, 116, 1, NULL),
(20, 117, 1, NULL),
(20, 118, 1, NULL),
(20, 119, 1, NULL),
(20, 120, 1, NULL),
(20, 121, 1, NULL),
(20, 122, 1, NULL),
(20, 123, 1, NULL),
(21, 114, 1, NULL),
(21, 113, 1, NULL),
(21, 112, 1, NULL),
(21, 95, 1, NULL),
(21, 94, 1, NULL),
(21, 93, 1, NULL),
(21, 124, 1, NULL),
(19, 94, 1, NULL),
(19, 93, 1, NULL);
-- --------------------------------------------------------
--
-- 表的结构 `fz_cart`
--
CREATE TABLE `fz_cart` (
`id` int(10) UNSIGNED NOT NULL,
`user_id` int(10) UNSIGNED NOT NULL,
`product_id` int(10) UNSIGNED NOT NULL,
`addtime` int(11) NOT NULL,
`addIP` int(11) NOT NULL,
`remark` varchar(255) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- 表的结构 `fz_node`
--
CREATE TABLE `fz_node` (
`id` smallint(6) UNSIGNED NOT NULL,
`name` varchar(20) NOT NULL,
`title` varchar(50) DEFAULT NULL,
`status` tinyint(1) DEFAULT '0',
`remark` varchar(255) DEFAULT NULL,
`sort` smallint(6) UNSIGNED DEFAULT NULL,
`pid` smallint(6) UNSIGNED NOT NULL,
`level` tinyint(1) UNSIGNED NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_node`
--
INSERT INTO `fz_node` (`id`, `name`, `title`, `status`, `remark`, `sort`, `pid`, `level`) VALUES
(93, 'Admin', NULL, 1, '后台应用', NULL, 0, 1),
(94, 'Index', NULL, 1, '后台主页', NULL, 93, 2),
(95, 'index', NULL, 1, '后台主页页面', NULL, 94, 3),
(96, 'Role', NULL, 1, '角色管理', NULL, 93, 2),
(97, 'index', NULL, 1, '角色管理页面', NULL, 96, 3),
(98, 'add', NULL, 1, '添加管理角色', NULL, 96, 3),
(99, 'del', NULL, 1, '删除管理角色', NULL, 96, 3),
(100, 'status', NULL, 1, '改变角色状态', NULL, 96, 3),
(101, 'Node', NULL, 1, '节点管理', NULL, 93, 2),
(102, 'index', NULL, 1, '节点管理页面', NULL, 101, 3),
(103, 'add', NULL, 1, '添加网站节点', NULL, 101, 3),
(104, 'del', NULL, 1, '删除网站节点', NULL, 101, 3),
(105, 'edit', NULL, 1, '修改网站节点', NULL, 101, 3),
(106, 'Access', NULL, 1, '权限设置', NULL, 93, 2),
(107, 'index', NULL, 1, '权限设置页面', NULL, 106, 3),
(108, 'setAccess', NULL, 1, '设置角色权限', NULL, 106, 3),
(109, 'Verify', NULL, 1, '申述用户权限', NULL, 93, 2),
(110, 'index', NULL, 1, '申核用户权限页面', NULL, 109, 3),
(111, 'verify', NULL, 1, '申核用户权限', NULL, 109, 3),
(112, 'Product', NULL, 1, '商品管理', NULL, 93, 2),
(113, 'index', NULL, 1, '商品管理页面', NULL, 112, 3),
(114, 'add', NULL, 1, '添加商品', NULL, 112, 3),
(116, 'Attr', NULL, 1, '商品属性', NULL, 93, 2),
(117, 'index', NULL, 1, '商品属性页面', NULL, 116, 3),
(118, 'add', NULL, 1, '添加商品属性', NULL, 116, 3),
(119, 'del', NULL, 1, '删除商品属性', NULL, 116, 3),
(120, 'Type', NULL, 1, '商品分类', NULL, 93, 2),
(121, 'index', NULL, 1, '商品分类页面', NULL, 120, 3),
(122, 'add', NULL, 1, '添加商品分类', NULL, 120, 3),
(123, 'del', NULL, 1, '删除商品分类', NULL, 120, 3),
(124, 'find', NULL, 1, '查找商品', NULL, 112, 3),
(125, 'Home', NULL, 1, '前台应用', NULL, 0, 1);
-- --------------------------------------------------------
--
-- 表的结构 `fz_pattr`
--
CREATE TABLE `fz_pattr` (
`id` int(10) UNSIGNED NOT NULL,
`name` varchar(20) DEFAULT NULL,
`color` varchar(10) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_pattr`
--
INSERT INTO `fz_pattr` (`id`, `name`, `color`) VALUES
(47, '热销', '#FF0000'),
(48, '包邮', '#2F9F00'),
(49, '7天退货', '#0000FF'),
(51, '8折', '#FF00FF');
-- --------------------------------------------------------
--
-- 表的结构 `fz_product`
--
CREATE TABLE `fz_product` (
`id` int(11) UNSIGNED NOT NULL,
`ptype_id` int(11) UNSIGNED NOT NULL,
`name` varchar(50) NOT NULL,
`price` float NOT NULL,
`describe` text NOT NULL,
`addtime` int(11) NOT NULL,
`shopcount` int(11) NOT NULL,
`shop_id` int(11) NOT NULL,
`shopname` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_product`
--
INSERT INTO `fz_product` (`id`, `ptype_id`, `name`, `price`, `describe`, `addtime`, `shopcount`, `shop_id`, `shopname`) VALUES
(57, 28, '宏碁V5-552G笔记本', 2988, '宏碁 V5-552G四核独显笔记本电脑宏碁 V5-552G四核独显笔记本电脑宏碁 V5-552G四核独显笔记本电脑宏碁 V5-552G四核独显笔记本电脑宏碁 V5-552G四核独显笔记本电脑宏碁 V5-552G四核独显笔记本电脑', 1462902306, 0, 26, '华翔宏基专卖店'),
(58, 29, '宏基超薄显示器', 1088, '宏基超薄显示器', 1462902496, 0, 26, '华翔宏基专卖店'),
(59, 26, '华硕P8H61PLUS', 279, 'Asus/华硕P8H61PLUS电脑主板LGA1155主板拼H61M H67 全国包邮 ', 1463146784, 0, 26, '华翔宏基专卖店'),
(60, 26, '技嘉 B85M-D3V-A', 429, 'Gigabyte/技嘉 B85M-D3V-A台式机B85电脑主板支持I3 4170 I5 4590 ', 1463149497, 0, 26, '华翔宏基专卖店'),
(61, 26, '技嘉 GA-F2A78M-DS2 FM2', 379, 'Gigabyte/技嘉 GA-F2A78M-DS2 FM2+主板 全固态电容 支持FM2 FM2+ ', 1463149609, 0, 26, '华翔宏基专卖店'),
(62, 26, '七彩虹 C.H81M V23', 259, 'Colorful/七彩虹 C.H81M V23 升级为V24 全固态小主板 支持G3260 全日系 固态电容\r\n', 1463149744, 0, 26, '华翔宏基专卖店'),
(63, 26, '华擎科技 Z170 Pro4S 1151', 699, 'ASROCK/华擎科技 Z170 Pro4S 1151主板 6400T非K版超频 十项供电 顺丰包邮 数字供 电,大板 双卡交火\r\n', 1463149857, 0, 26, '华翔宏基专卖店'),
(64, 27, '精影 GTX650 1G', 388, '精影 GTX650 1G高端电脑显卡D5高频比R7-350 HD6850强 促销抢购! 高频高性能 稳定低功耗 高性价比\r\n', 1463150057, 0, 26, '华翔宏基专卖店');
-- --------------------------------------------------------
--
-- 表的结构 `fz_product_imgurl`
--
CREATE TABLE `fz_product_imgurl` (
`product_id` int(11) NOT NULL,
`imgurl` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_product_imgurl`
--
INSERT INTO `fz_product_imgurl` (`product_id`, `imgurl`) VALUES
(52, '/UpLoads/product/images/2016-05-05/572a4eb87c6b7.jpg'),
(53, '/UpLoads/product/images/2016-05-05/572a50593da2c.jpg'),
(54, '/UpLoads/product/images/2016-05-05/572b1172825e0.jpg'),
(55, '/UpLoads/product/images/2016-05-05/572b1b8b3e74e.jpg'),
(56, '/UpLoads/product/images/2016-05-05/572b1bcbf30c5.jpg'),
(57, '/UpLoads/product/images/2016-05-11/57321e2280412.jpg'),
(58, '/UpLoads/product/images/2016-05-11/57321ee01f896.jpg'),
(59, '/UpLoads/product/images/2016-05-13/5735d9208ec5d.png'),
(60, '/UpLoads/product/images/2016-05-13/5735e3b9cfb9a.jpg'),
(61, '/UpLoads/product/images/2016-05-13/5735e4296426e.jpg'),
(62, '/UpLoads/product/images/2016-05-13/5735e4b016716.jpg'),
(63, '/UpLoads/product/images/2016-05-13/5735e521489b5.jpg'),
(64, '/UpLoads/product/images/2016-05-13/5735e5e96ecce.jpg');
-- --------------------------------------------------------
--
-- 表的结构 `fz_product_pattr`
--
CREATE TABLE `fz_product_pattr` (
`product_id` int(11) DEFAULT NULL,
`pattr_id` int(11) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_product_pattr`
--
INSERT INTO `fz_product_pattr` (`product_id`, `pattr_id`) VALUES
(57, 49),
(57, 47),
(58, 47),
(58, 48),
(58, 49),
(58, 51),
(65, 49),
(59, 47),
(59, 48),
(59, 51),
(60, 48),
(60, 51),
(61, 47),
(61, 49),
(62, 47),
(62, 48),
(62, 49),
(63, 47),
(63, 48),
(63, 51),
(64, 47),
(64, 48);
-- --------------------------------------------------------
--
-- 表的结构 `fz_ptype`
--
CREATE TABLE `fz_ptype` (
`id` int(11) UNSIGNED NOT NULL,
`pid` int(11) NOT NULL DEFAULT '0',
`ptype_name` varchar(20) NOT NULL,
`order` int(11) NOT NULL DEFAULT '0'
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_ptype`
--
INSERT INTO `fz_ptype` (`id`, `pid`, `ptype_name`, `order`) VALUES
(26, 0, '主板', 100),
(27, 0, '显卡', 100),
(28, 0, '笔记本', 100),
(29, 0, '显示器', 100),
(30, 0, '内存条', 100),
(31, 0, '网络设备', 100);
-- --------------------------------------------------------
--
-- 表的结构 `fz_role`
--
CREATE TABLE `fz_role` (
`id` smallint(6) UNSIGNED NOT NULL,
`name` varchar(20) NOT NULL,
`pid` smallint(6) DEFAULT NULL,
`status` tinyint(1) UNSIGNED DEFAULT NULL,
`remark` varchar(255) DEFAULT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_role`
--
INSERT INTO `fz_role` (`id`, `name`, `pid`, `status`, `remark`) VALUES
(19, 'admin', NULL, 1, '超级管理员'),
(20, 'HQ', NULL, 1, '总店管理员'),
(21, 'ST', NULL, 1, '分店管理员');
-- --------------------------------------------------------
--
-- 表的结构 `fz_role_user`
--
CREATE TABLE `fz_role_user` (
`role_id` mediumint(9) UNSIGNED DEFAULT NULL,
`user_id` char(32) DEFAULT NULL
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_role_user`
--
INSERT INTO `fz_role_user` (`role_id`, `user_id`) VALUES
(20, '18'),
(19, '19'),
(20, '20'),
(21, '21'),
(21, '24'),
(21, '22'),
(21, '25'),
(21, '26'),
(21, '27'),
(21, '28'),
(21, '29'),
(21, '30');
-- --------------------------------------------------------
--
-- 表的结构 `fz_shop`
--
CREATE TABLE `fz_shop` (
`id` int(11) UNSIGNED NOT NULL,
`shopname` varchar(255) NOT NULL,
`peoplename` varchar(20) NOT NULL,
`loginword` varchar(255) NOT NULL,
`peoplephone` varchar(11) NOT NULL,
`shopphone` varchar(11) NOT NULL,
`address` varchar(255) NOT NULL,
`regtime` int(11) NOT NULL,
`regIP` varchar(255) NOT NULL,
`countshop` bigint(11) DEFAULT NULL,
`countmoney` bigint(11) DEFAULT NULL,
`level` int(11) DEFAULT NULL,
`token` varchar(255) DEFAULT NULL,
`describe` text NOT NULL,
`lock` tinyint(1) DEFAULT '1',
`type` tinyint(4) NOT NULL DEFAULT '1'
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_shop`
--
INSERT INTO `fz_shop` (`id`, `shopname`, `peoplename`, `loginword`, `peoplephone`, `shopphone`, `address`, `regtime`, `regIP`, `countshop`, `countmoney`, `level`, `token`, `describe`, `lock`, `type`) VALUES
(18, 'flyzebra', 'flyzebra', '10e208ffd957f5488f795e555f483af8', '13520989725', '13520989725', '广西桂林资源县', 1462386990, '192.168.1.88', NULL, NULL, NULL, NULL, '总店管理号。', 1, 0),
(19, 'admin', 'admin', '10e208ffd957f5488f795e555f483af8', '13520989725', '13520989725', '1', 1462388077, '192.168.1.88', NULL, NULL, NULL, NULL, '1', 1, 0),
(20, 'hq', 'hq', '10e208ffd957f5488f795e555f483af8', '13520989725', '13520989725', 'hq', 1462388281, '192.168.1.88', NULL, NULL, NULL, NULL, 'hq', 1, 0),
(21, 'st', 'st', '10e208ffd957f5488f795e555f483af8', '13520989725', '13520989725', 'st', 1462388307, '192.168.1.88', NULL, NULL, NULL, NULL, 'st', 1, 0),
(26, '华翔宏基专卖店', '张三', '10e208ffd957f5488f795e555f483af8', '13912345678', '13912345678', '1391234567813912345678139123456781391234567813912', 1462814854, '192.168.1.88', NULL, NULL, NULL, NULL, '139123456781391234567813912345678139123456781391234', 1, 1),
(27, '卓越联想品牌店', '李四', '10e208ffd957f5488f795e555f483af8', '13912345678', '13912345678', '13912345678139123456781391234567813912345678139123', 1462814942, '192.168.1.88', NULL, NULL, NULL, NULL, '1391234567813912345678139123456781391234567813', 1, 1),
(28, '联想笔记本专卖店', '王五', '10e208ffd957f5488f795e555f483af8', '13812345678', '13812345678', '1381234567813812345678138123456781381234567813812345678', 1462817151, '192.168.1.88', NULL, NULL, NULL, NULL, '138123456781381234567813812345678138123456', 1, 1),
(29, '新艺华硕专卖店旗舰店', '赵六', '10e208ffd957f5488f795e555f483af8', '13512345678', '13512345678', '1351234567813512345678135123456781351234567813512345678', 1462871674, '192.168.1.88', NULL, NULL, NULL, NULL, '13512345678135123456781351234567813512345678135123456781351234567813512345678', 1, 1),
(30, '飞马Dell专卖店', '飞马', '10e208ffd957f5488f795e555f483af8', '13612345678', '13612345678', '13612345678136123456781361234567813612345678', 1462871887, '192.168.1.88', NULL, NULL, NULL, NULL, '13612345678136123456781361234567813612345678136123456781361234567813612345678', 1, 1);
-- --------------------------------------------------------
--
-- 表的结构 `fz_shop_home`
--
CREATE TABLE `fz_shop_home` (
`shop_id` int(10) UNSIGNED NOT NULL,
`show` tinyint(1) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_shop_home`
--
INSERT INTO `fz_shop_home` (`shop_id`, `show`) VALUES
(26, 1),
(27, 1),
(28, 1),
(29, 1),
(30, 1);
-- --------------------------------------------------------
--
-- 表的结构 `fz_shop_imgurl`
--
CREATE TABLE `fz_shop_imgurl` (
`shop_id` int(11) NOT NULL,
`imgurl` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_shop_imgurl`
--
INSERT INTO `fz_shop_imgurl` (`shop_id`, `imgurl`) VALUES
(26, '/UpLoads/shop/images/2016-05-10/5730c886e6755.jpg'),
(27, '/UpLoads/shop/images/2016-05-10/5730c8dec92b0.jpg'),
(28, '/UpLoads/shop/images/2016-05-10/5730d17fd3fee.jpg'),
(29, '/UpLoads/shop/images/2016-05-10/5731a67a6e31e.jpg'),
(30, '/UpLoads/shop/images/2016-05-10/5731a74f58058.jpg');
-- --------------------------------------------------------
--
-- 表的结构 `fz_shop_log`
--
CREATE TABLE `fz_shop_log` (
`shop_id` int(11) NOT NULL,
`loginIP` varchar(32) NOT NULL,
`logintime` int(11) NOT NULL DEFAULT '0',
`gps` varchar(255) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_shop_log`
--
INSERT INTO `fz_shop_log` (`shop_id`, `loginIP`, `logintime`, `gps`) VALUES
(18, '192.168.1.88', 1462855612, NULL),
(18, '192.168.1.88', 1462870725, NULL),
(18, '192.168.1.88', 1462871038, NULL),
(18, '192.168.1.88', 1462871164, NULL),
(18, '192.168.1.88', 1462871165, NULL),
(26, '192.168.1.88', 1462899294, NULL),
(26, '192.168.1.88', 1462899305, NULL),
(18, '192.168.1.88', 1462899314, NULL),
(26, '192.168.1.88', 1462899355, NULL),
(26, '192.168.1.88', 1462900958, NULL),
(18, '192.168.1.88', 1462901013, NULL),
(26, '192.168.1.88', 1462902179, NULL),
(18, '192.168.1.88', 1462902416, NULL),
(26, '192.168.1.88', 1462902432, NULL),
(26, '192.168.1.88', 1463039178, NULL),
(26, '192.168.1.88', 1463062535, NULL),
(18, '192.168.1.88', 1463063644, NULL),
(18, '192.168.1.88', 1463120986, NULL),
(18, '192.168.1.88', 1463127837, NULL),
(26, '192.168.1.88', 1463146379, NULL),
(26, '192.168.1.88', 1463337194, NULL),
(18, '192.168.1.88', 1463337202, NULL),
(26, '192.168.1.88', 1463380320, NULL),
(18, '192.168.1.88', 1463926825, NULL),
(18, '192.168.1.88', 1466081243, NULL),
(18, '192.168.1.88', 1466228749, NULL),
(18, '192.168.1.88', 1466262514, NULL),
(18, '192.168.1.88', 1468335507, NULL),
(18, '192.168.1.88', 1494329341, NULL),
(18, '192.168.1.188', 1521349635, NULL),
(18, '192.168.1.188', 1521349655, NULL);
-- --------------------------------------------------------
--
-- 表的结构 `fz_user`
--
CREATE TABLE `fz_user` (
`id` int(11) UNSIGNED NOT NULL,
`loginname` varchar(20) DEFAULT NULL,
`nickname` varchar(20) NOT NULL,
`loginword` varchar(255) DEFAULT NULL,
`telephone` varchar(20) DEFAULT NULL,
`openid` varchar(255) DEFAULT NULL,
`loginwith` varchar(10) DEFAULT NULL,
`pictureurl` varchar(255) DEFAULT NULL,
`address` varchar(255) DEFAULT NULL,
`level` int(11) NOT NULL,
`logincount` int(11) NOT NULL,
`regtime` int(11) NOT NULL,
`token` varchar(255) DEFAULT NULL,
`lock` tinyint(4) DEFAULT '1'
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_user`
--
INSERT INTO `fz_user` (`id`, `loginname`, `nickname`, `loginword`, `telephone`, `openid`, `loginwith`, `pictureurl`, `address`, `level`, `logincount`, `regtime`, `token`, `lock`) VALUES
(112, 'flyzebra', 'flyzebra', '<PASSWORD>', NULL, NULL, NULL, NULL, NULL, 10, 56, 1462379129, NULL, 1),
(113, 'flyzebraa', 'flyzebraa', '10e208ffd957f5488f795e555f483af8', NULL, NULL, NULL, NULL, NULL, 1, 1, 1462384698, NULL, 1),
(114, NULL, 'FlyZebra', NULL, NULL, '7DDCD68540AA1B4D3C02FF65D8A0935F', 'QQ', 'http://q.qlogo.cn/qqapp/1105211644/7DDCD68540AA1B4D3C02FF65D8A0935F/100', NULL, 10, 57, 1462392826, NULL, 1),
(115, NULL, '尋找紅葉', NULL, NULL, '252027078', 'BAIDU', 'http://himg.bdimg.com/sys/portraitn/item/5a70e5b08be689bee7b485e89189214f.jpg', NULL, 12, 93, 1462392844, NULL, 1),
(116, 'ff', 'ff', '83be264eb452fcf0a1c322f2c7cbf987', NULL, NULL, NULL, NULL, NULL, 3, 6, 1462642658, NULL, 1),
(117, NULL, '宇翔科技', 'd41d8cd98f00b204e9800998ecf8427e', NULL, '2035EB7D382C45688D1AB390F199F9D2', 'QQ', 'http://q.qlogo.cn/qqapp/1105211644/2035EB7D382C45688D1AB390F199F9D2/100', NULL, 9, 46, 1462644768, NULL, 1),
(118, 'tsy', 'tsy', '<PASSWORD>', NULL, NULL, NULL, NULL, NULL, 11, 73, 1463055990, NULL, 1),
(119, 'tyty', 'tyty', '<PASSWORD>', NULL, NULL, NULL, NULL, NULL, 8, 33, 1463227403, NULL, 1);
-- --------------------------------------------------------
--
-- 表的结构 `fz_user_car`
--
CREATE TABLE `fz_user_car` (
`user_id` int(10) UNSIGNED NOT NULL,
`product_id` int(10) UNSIGNED NOT NULL,
`addtime` int(11) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- 表的结构 `fz_user_copy`
--
CREATE TABLE `fz_user_copy` (
`id` int(11) UNSIGNED NOT NULL,
`loginname` varchar(20) DEFAULT NULL,
`nickname` varchar(20) NOT NULL,
`loginword` varchar(255) DEFAULT NULL,
`telephone` varchar(20) DEFAULT NULL,
`openid` varchar(255) DEFAULT NULL,
`loginwith` varchar(10) DEFAULT NULL,
`pictureurl` varchar(255) DEFAULT NULL,
`address` varchar(255) DEFAULT NULL,
`level` int(11) NOT NULL,
`logincount` int(11) NOT NULL,
`regtime` int(11) NOT NULL,
`token` varchar(255) DEFAULT NULL,
`lock` tinyint(4) DEFAULT '1'
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_user_copy`
--
INSERT INTO `fz_user_copy` (`id`, `loginname`, `nickname`, `loginword`, `telephone`, `openid`, `loginwith`, `pictureurl`, `address`, `level`, `logincount`, `regtime`, `token`, `lock`) VALUES
(112, 'flyzebra', 'flyzebra', '<PASSWORD>', NULL, NULL, NULL, NULL, NULL, 10, 56, 1462379129, NULL, 1),
(113, 'flyzebraa', 'flyzebraa', '10e208ffd957f5488f795e555f483af8', NULL, NULL, NULL, NULL, NULL, 1, 1, 1462384698, NULL, 1),
(114, NULL, 'FlyZebra', NULL, NULL, '7DDCD68540AA1B4D3C02FF65D8A0935F', 'QQ', 'http://q.qlogo.cn/qqapp/1105211644/7DDCD68540AA1B4D3C02FF65D8A0935F/100', NULL, 10, 57, 1462392826, NULL, 1),
(115, NULL, '尋找紅葉', NULL, NULL, '252027078', 'BAIDU', 'http://himg.bdimg.com/sys/portraitn/item/5a70e5b08be689bee7b485e89189214f.jpg', NULL, 12, 93, 1462392844, NULL, 1),
(116, 'ff', 'ff', '83be264eb452fcf0a1c322f2c7cbf987', NULL, NULL, NULL, NULL, NULL, 3, 6, 1462642658, NULL, 1),
(117, NULL, '宇翔科技', 'd41d8cd98f00b204e9800998ecf8427e', NULL, '2035EB7D382C45688D1AB390F199F9D2', 'QQ', 'http://q.qlogo.cn/qqapp/1105211644/2035EB7D382C45688D1AB390F199F9D2/100', NULL, 9, 46, 1462644768, NULL, 1),
(118, 'tsy', 'tsy', 'b5d2099e49bdb07b8176dff5e23b3c14', NULL, NULL, NULL, NULL, NULL, 11, 73, 1463055990, NULL, 1),
(119, 'tyty', 'tyty', 'b5d2099e49bdb07b8176dff5e23b3c14', NULL, NULL, NULL, NULL, NULL, 8, 33, 1463227403, NULL, 1);
-- --------------------------------------------------------
--
-- 表的结构 `fz_user_log`
--
CREATE TABLE `fz_user_log` (
`user_id` int(11) NOT NULL,
`loginIP` varchar(32) NOT NULL,
`logintime` int(11) NOT NULL,
`gps` varchar(255) DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_user_log`
--
INSERT INTO `fz_user_log` (`user_id`, `loginIP`, `logintime`, `gps`) VALUES
(112, '192.168.1.88', 1462379129, NULL),
(112, '192.168.1.88', 1462381561, NULL),
(112, '192.168.1.88', 1462381873, NULL),
(112, '192.168.1.88', 1462382000, NULL),
(112, '192.168.1.88', 1462382067, NULL),
(112, '192.168.1.88', 1462382107, NULL),
(112, '192.168.1.88', 1462382116, NULL),
(112, '192.168.1.88', 1462382250, NULL),
(112, '192.168.1.88', 1462383068, NULL),
(112, '192.168.1.88', 1462383080, NULL),
(112, '192.168.1.88', 1462383203, NULL),
(112, '192.168.1.88', 1462383208, NULL),
(112, '192.168.1.88', 1462383275, NULL),
(112, '192.168.1.88', 1462383310, NULL),
(112, '192.168.1.88', 1462383404, NULL),
(112, '192.168.1.88', 1462384471, NULL),
(113, '192.168.1.88', 1462384698, NULL),
(112, '192.168.1.88', 1462436330, NULL),
(115, '192.168.1.105', 1462636116, NULL),
(115, '192.168.1.105', 1462636569, NULL),
(115, '192.168.1.105', 1462638400, NULL),
(115, '192.168.1.105', 1462638415, NULL),
(115, '192.168.1.105', 1462638437, NULL),
(115, '192.168.1.105', 1462638547, NULL),
(115, '192.168.1.105', 1462638591, NULL),
(115, '192.168.1.105', 1462638608, NULL),
(115, '192.168.1.105', 1462639984, NULL),
(115, '192.168.1.105', 1462642638, NULL),
(116, '192.168.1.105', 1462642658, NULL),
(116, '192.168.1.105', 1462643677, NULL),
(114, '192.168.1.105', 1462643687, NULL),
(114, '192.168.1.105', 1462643707, NULL),
(116, '192.168.1.105', 1462643722, NULL),
(116, '192.168.1.105', 1462643882, NULL),
(114, '192.168.1.105', 1462643923, NULL),
(114, '192.168.1.105', 1462643929, NULL),
(114, '192.168.1.105', 1462644574, NULL),
(114, '192.168.1.105', 1462644638, NULL),
(114, '192.168.1.105', 1462644647, NULL),
(114, '192.168.1.105', 1462644674, NULL),
(114, '192.168.1.105', 1462644710, NULL),
(115, '192.168.1.105', 1462644738, NULL),
(115, '192.168.1.105', 1462644749, NULL),
(115, '192.168.1.105', 1462644754, NULL),
(117, '192.168.1.105', 1462644768, NULL),
(116, '192.168.1.105', 1462644872, NULL),
(116, '192.168.1.105', 1462645351, NULL),
(117, '192.168.1.105', 1462645363, NULL),
(117, '192.168.1.105', 1462645375, NULL),
(117, '192.168.1.105', 1462645592, NULL),
(117, '192.168.1.105', 1462653702, NULL),
(117, '192.168.1.105', 1462683891, NULL),
(117, '192.168.1.105', 1462691821, NULL),
(117, '192.168.1.105', 1462701610, NULL),
(117, '192.168.1.105', 1462701881, NULL),
(117, '192.168.1.105', 1462702014, NULL),
(117, '192.168.1.105', 1462702854, NULL),
(117, '192.168.1.105', 1462703582, NULL),
(117, '192.168.1.105', 1462703609, NULL),
(117, '192.168.1.105', 1462703928, NULL),
(117, '192.168.1.105', 1462703965, NULL),
(117, '192.168.1.105', 1462704003, NULL),
(117, '192.168.1.105', 1462704062, NULL),
(117, '192.168.1.105', 1462705214, NULL),
(117, '192.168.1.105', 1462705455, NULL),
(117, '192.168.1.105', 1462716437, NULL),
(117, '192.168.1.105', 1462717249, NULL),
(117, '192.168.1.105', 1462718674, NULL),
(117, '192.168.1.105', 1462718720, NULL),
(117, '192.168.1.105', 1462718919, NULL),
(117, '192.168.1.105', 1462719153, NULL),
(117, '192.168.1.105', 1462719513, NULL),
(117, '192.168.1.105', 1462725445, NULL),
(117, '192.168.1.105', 1462783214, NULL),
(117, '192.168.1.105', 1462793501, NULL),
(117, '192.168.1.105', 1462794686, NULL),
(117, '192.168.1.105', 1462794711, NULL),
(117, '192.168.1.105', 1462795640, NULL),
(117, '192.168.1.105', 1462798778, NULL),
(117, '192.168.1.105', 1462812393, NULL),
(117, '192.168.1.105', 1462812596, NULL),
(117, '192.168.1.105', 1462812800, NULL),
(117, '192.168.1.105', 1462812918, NULL),
(117, '192.168.1.105', 1462813635, NULL),
(115, '192.168.1.105', 1462813694, NULL),
(115, '192.168.1.105', 1462859183, NULL),
(115, '192.168.1.105', 1462873631, NULL),
(115, '192.168.1.105', 1462874227, NULL),
(115, '192.168.1.105', 1462874791, NULL),
(115, '192.168.1.105', 1462875466, NULL),
(115, '192.168.1.105', 1462875511, NULL),
(115, '192.168.1.105', 1462875530, NULL),
(115, '192.168.1.105', 1462875666, NULL),
(115, '192.168.1.105', 1462875926, NULL),
(115, '192.168.1.105', 1462876170, NULL),
(115, '192.168.1.105', 1462876216, NULL),
(117, '192.168.1.105', 1463055885, NULL),
(117, '192.168.1.105', 1463055961, NULL),
(118, '192.168.1.105', 1463055990, NULL),
(118, '192.168.1.105', 1463057803, NULL),
(118, '192.168.1.105', 1463059265, NULL),
(118, '192.168.1.105', 1463059426, NULL),
(118, '192.168.1.105', 1463059824, NULL),
(118, '192.168.1.105', 1463059917, NULL),
(118, '192.168.1.105', 1463061938, NULL),
(118, '192.168.1.105', 1463062255, NULL),
(118, '192.168.1.105', 1463062366, NULL),
(118, '192.168.1.105', 1463062466, NULL),
(118, '192.168.1.105', 1463062693, NULL),
(118, '192.168.1.105', 1463062799, NULL),
(118, '192.168.1.105', 1463063003, NULL),
(118, '192.168.1.105', 1463063218, NULL),
(118, '192.168.1.105', 1463063319, NULL),
(118, '192.168.1.105', 1463063506, NULL),
(118, '192.168.1.105', 1463063789, NULL),
(118, '192.168.1.105', 1463063937, NULL),
(118, '192.168.1.105', 1463063991, NULL),
(118, '192.168.1.105', 1463064050, NULL),
(118, '192.168.1.105', 1463064474, NULL),
(118, '192.168.1.105', 1463065025, NULL),
(118, '192.168.1.105', 1463065466, NULL),
(118, '192.168.1.105', 1463065489, NULL),
(118, '192.168.1.105', 1463065948, NULL),
(118, '192.168.1.105', 1463065962, NULL),
(118, '192.168.1.105', 1463065977, NULL),
(118, '192.168.1.105', 1463066003, NULL),
(118, '192.168.1.105', 1463067759, NULL),
(118, '192.168.1.105', 1463069517, NULL),
(118, '192.168.1.105', 1463071182, NULL),
(118, '192.168.1.105', 1463073300, NULL),
(118, '192.168.1.105', 1463117814, NULL),
(118, '192.168.1.105', 1463118837, NULL),
(118, '192.168.1.105', 1463118877, NULL),
(118, '192.168.1.105', 1463119907, NULL),
(118, '192.168.1.105', 1463121048, NULL),
(118, '192.168.1.105', 1463121450, NULL),
(118, '192.168.1.105', 1463122154, NULL),
(118, '192.168.1.105', 1463124426, NULL),
(118, '192.168.1.105', 1463124497, NULL),
(118, '192.168.1.105', 1463124703, NULL),
(118, '192.168.1.105', 1463125825, NULL),
(118, '192.168.1.105', 1463127486, NULL),
(118, '192.168.1.105', 1463127552, NULL),
(118, '192.168.1.105', 1463127578, NULL),
(118, '192.168.1.105', 1463127744, NULL),
(118, '192.168.1.105', 1463127910, NULL),
(118, '192.168.1.105', 1463127992, NULL),
(118, '192.168.1.105', 1463128150, NULL),
(118, '192.168.1.105', 1463129210, NULL),
(118, '192.168.1.105', 1463129379, NULL),
(118, '192.168.1.105', 1463129478, NULL),
(118, '192.168.1.105', 1463129581, NULL),
(118, '192.168.1.105', 1463129761, NULL),
(118, '192.168.1.105', 1463130136, NULL),
(118, '192.168.1.105', 1463130164, NULL),
(118, '192.168.1.105', 1463130341, NULL),
(118, '192.168.1.105', 1463130600, NULL),
(118, '192.168.1.105', 1463130707, NULL),
(118, '192.168.1.105', 1463131024, NULL),
(118, '192.168.1.105', 1463131152, NULL),
(118, '192.168.1.105', 1463131217, NULL),
(118, '192.168.1.105', 1463131529, NULL),
(118, '192.168.1.105', 1463131780, NULL),
(118, '192.168.1.105', 1463131843, NULL),
(118, '192.168.1.105', 1463132001, NULL),
(118, '192.168.1.105', 1463132032, NULL),
(118, '192.168.1.105', 1463132103, NULL),
(118, '192.168.1.105', 1463132268, NULL),
(118, '192.168.1.105', 1463132370, NULL),
(118, '192.168.1.105', 1463132503, NULL),
(118, '192.168.1.105', 1463132633, NULL),
(117, '192.168.1.105', 1463158561, NULL),
(117, '192.168.1.105', 1463158580, NULL),
(117, '192.168.1.105', 1463158597, NULL),
(117, '192.168.1.105', 1463158930, NULL),
(117, '192.168.1.105', 1463158999, NULL),
(115, '192.168.1.105', 1463159089, NULL),
(115, '192.168.1.105', 1463159114, NULL),
(115, '192.168.1.105', 1463159375, NULL),
(115, '192.168.1.105', 1463159537, NULL),
(115, '192.168.1.105', 1463159709, NULL),
(115, '192.168.1.105', 1463159761, NULL),
(115, '192.168.1.105', 1463160048, NULL),
(115, '192.168.1.105', 1463160394, NULL),
(115, '192.168.1.105', 1463160654, NULL),
(115, '192.168.1.105', 1463160844, NULL),
(115, '192.168.1.105', 1463161449, NULL),
(115, '192.168.1.105', 1463161518, NULL),
(115, '192.168.1.105', 1463161584, NULL),
(115, '192.168.1.105', 1463161687, NULL),
(115, '192.168.1.105', 1463162601, NULL),
(115, '192.168.1.105', 1463168996, NULL),
(115, '192.168.1.105', 1463169033, NULL),
(115, '192.168.1.105', 1463197217, NULL),
(115, '192.168.1.105', 1463197277, NULL),
(115, '192.168.1.105', 1463197468, NULL),
(115, '192.168.1.105', 1463202042, NULL),
(115, '192.168.1.105', 1463202707, NULL),
(115, '192.168.1.105', 1463203925, NULL),
(115, '192.168.1.105', 1463206311, NULL),
(115, '192.168.1.105', 1463208996, NULL),
(115, '192.168.1.105', 1463218119, NULL),
(115, '192.168.1.105', 1463218128, NULL),
(115, '192.168.1.105', 1463218676, NULL),
(115, '192.168.1.105', 1463218797, NULL),
(115, '192.168.1.105', 1463218873, NULL),
(115, '192.168.1.105', 1463219009, NULL),
(115, '192.168.1.105', 1463219063, NULL),
(115, '192.168.1.105', 1463219653, NULL),
(115, '192.168.1.105', 1463220188, NULL),
(115, '192.168.1.105', 1463220338, NULL),
(115, '192.168.1.105', 1463220482, NULL),
(115, '192.168.1.105', 1463220571, NULL),
(115, '192.168.1.105', 1463223075, NULL),
(115, '192.168.1.105', 1463223375, NULL),
(115, '192.168.1.105', 1463223410, NULL),
(115, '192.168.1.105', 1463223439, NULL),
(115, '192.168.1.105', 1463223774, NULL),
(115, '192.168.1.105', 1463223997, NULL),
(115, '192.168.1.105', 1463224435, NULL),
(115, '192.168.1.105', 1463225079, NULL),
(115, '192.168.1.105', 1463226578, NULL),
(115, '192.168.1.105', 1463226824, NULL),
(115, '192.168.1.105', 1463226832, NULL),
(115, '192.168.1.105', 1463226853, NULL),
(115, '192.168.1.105', 1463227153, NULL),
(115, '192.168.1.105', 1463227171, NULL),
(117, '192.168.1.105', 1463227184, NULL),
(112, '192.168.1.105', 1463227238, NULL),
(112, '192.168.1.105', 1463227263, NULL),
(112, '192.168.1.105', 1463227305, NULL),
(112, '192.168.1.105', 1463227328, NULL),
(112, '192.168.1.105', 1463227360, NULL),
(112, '192.168.1.105', 1463227380, NULL),
(119, '192.168.1.105', 1463227403, NULL),
(119, '192.168.1.105', 1463227422, NULL),
(119, '192.168.1.105', 1463228161, NULL),
(119, '192.168.1.105', 1463228196, NULL),
(119, '192.168.1.105', 1463228228, NULL),
(119, '192.168.1.105', 1463228299, NULL),
(119, '192.168.1.105', 1463228330, NULL),
(119, '192.168.1.105', 1463228631, NULL),
(119, '192.168.1.105', 1463229157, NULL),
(119, '192.168.1.105', 1463230653, NULL),
(119, '192.168.1.105', 1463230964, NULL),
(119, '192.168.1.105', 1463230984, NULL),
(119, '192.168.1.105', 1463231412, NULL),
(119, '192.168.1.105', 1463231542, NULL),
(119, '192.168.1.105', 1463231781, NULL),
(119, '192.168.1.105', 1463232382, NULL),
(119, '192.168.1.105', 1463232521, NULL),
(119, '192.168.1.105', 1463232801, NULL),
(119, '192.168.1.105', 1463232885, NULL),
(119, '192.168.1.105', 1463232954, NULL),
(119, '192.168.1.105', 1463233126, NULL),
(119, '192.168.1.105', 1463233157, NULL),
(119, '192.168.1.105', 1463233255, NULL),
(119, '192.168.1.105', 1463233809, NULL),
(119, '192.168.1.105', 1463234504, NULL),
(119, '192.168.1.105', 1463234516, NULL),
(119, '192.168.1.105', 1463234570, NULL),
(119, '192.168.1.105', 1463235243, NULL),
(119, '192.168.1.105', 1463235995, NULL),
(119, '192.168.1.105', 1463236097, NULL),
(119, '192.168.1.105', 1463236165, NULL),
(119, '192.168.1.105', 1463236276, NULL),
(119, '192.168.1.105', 1463236533, NULL),
(114, '192.168.1.105', 1463236614, NULL),
(114, '192.168.1.105', 1463236937, NULL),
(114, '192.168.1.105', 1463242771, NULL),
(112, '192.168.1.88', 1463245811, NULL),
(114, '192.168.1.105', 1463245900, NULL),
(112, '192.168.1.88', 1463246030, NULL),
(112, '192.168.1.88', 1463246186, NULL),
(112, '192.168.1.88', 1463246213, NULL),
(112, '192.168.1.88', 1463246339, NULL),
(114, '192.168.1.105', 1463246351, NULL),
(114, '192.168.1.105', 1463246359, NULL),
(112, '192.168.1.88', 1463246381, NULL),
(112, '192.168.1.88', 1463246395, NULL),
(112, '192.168.1.88', 1463246449, NULL),
(112, '192.168.1.88', 1463246682, NULL),
(112, '192.168.1.88', 1463246775, NULL),
(112, '192.168.1.88', 1463246839, NULL),
(112, '192.168.1.88', 1463246965, NULL),
(112, '192.168.1.88', 1463246981, NULL),
(112, '192.168.1.88', 1463247004, NULL),
(112, '192.168.1.88', 1463247023, NULL),
(112, '192.168.1.88', 1463247046, NULL),
(112, '192.168.1.88', 1463247133, NULL),
(112, '192.168.1.88', 1463247214, NULL),
(114, '192.168.1.105', 1463247361, NULL),
(114, '192.168.1.105', 1463247371, NULL),
(114, '192.168.1.105', 1463250533, NULL),
(114, '192.168.1.105', 1463250723, NULL),
(114, '192.168.1.105', 1463250951, NULL),
(114, '192.168.1.105', 1463251027, NULL),
(114, '192.168.1.105', 1463251086, NULL),
(114, '192.168.1.105', 1463251144, NULL),
(114, '192.168.1.105', 1463277686, NULL),
(114, '192.168.1.105', 1463277753, NULL),
(112, '192.168.1.88', 1463279765, NULL),
(112, '192.168.1.88', 1463279912, NULL),
(114, '192.168.1.105', 1463279982, NULL),
(114, '192.168.1.105', 1463280067, NULL),
(114, '192.168.1.105', 1463280098, NULL),
(114, '192.168.1.105', 1463280492, NULL),
(114, '192.168.1.105', 1463280626, NULL),
(112, '192.168.1.88', 1463280704, NULL),
(112, '192.168.1.88', 1463281017, NULL),
(112, '192.168.1.88', 1463281074, NULL),
(112, '192.168.1.88', 1463281153, NULL),
(114, '192.168.1.105', 1463281243, NULL),
(112, '192.168.1.88', 1463281299, NULL),
(114, '192.168.1.105', 1463281311, NULL),
(112, '192.168.1.88', 1463281351, NULL),
(114, '192.168.1.105', 1463281526, NULL),
(114, '192.168.1.105', 1463281596, NULL),
(112, '192.168.1.88', 1463282162, NULL),
(112, '192.168.1.88', 1463282260, NULL),
(112, '192.168.1.88', 1463282512, NULL),
(112, '192.168.1.88', 1463282618, NULL),
(112, '192.168.1.88', 1463282697, NULL),
(112, '192.168.1.88', 1463282785, NULL),
(114, '192.168.1.105', 1463282844, NULL),
(114, '192.168.1.105', 1463284093, NULL),
(114, '192.168.1.105', 1463284251, NULL),
(114, '192.168.1.105', 1463284355, NULL),
(114, '192.168.1.105', 1463286034, NULL),
(114, '192.168.1.105', 1463286194, NULL),
(114, '192.168.1.105', 1463287077, NULL),
(114, '192.168.1.105', 1463287266, NULL),
(114, '192.168.1.105', 1463288123, NULL),
(114, '192.168.1.105', 1463288452, NULL),
(114, '192.168.1.105', 1463291157, NULL),
(114, '192.168.1.105', 1463292627, NULL),
(114, '192.168.1.105', 1463292656, NULL),
(114, '192.168.1.105', 1463301487, NULL),
(114, '192.168.1.105', 1463308124, NULL),
(114, '192.168.1.105', 1463312644, NULL),
(114, '192.168.1.105', 1463319647, NULL),
(114, '192.168.1.105', 1463322407, NULL),
(115, '192.168.1.105', 1463477354, NULL),
(115, '192.168.1.105', 1463477464, NULL),
(115, '192.168.1.105', 1463477528, NULL),
(115, '192.168.1.105', 1463477602, NULL),
(115, '192.168.1.105', 1463477617, NULL),
(115, '192.168.1.105', 1463477800, NULL),
(115, '192.168.1.105', 1463477872, NULL),
(115, '192.168.1.105', 1463477934, NULL),
(115, '192.168.1.105', 1463477951, NULL),
(115, '192.168.1.105', 1463478186, NULL),
(115, '192.168.1.105', 1463479911, NULL),
(115, '192.168.1.105', 1463481011, NULL),
(112, '192.168.1.88', 1468028383, NULL),
(112, '192.168.1.88', 1468028415, NULL);
-- --------------------------------------------------------
--
-- 表的结构 `fz_welcome`
--
CREATE TABLE `fz_welcome` (
`id` int(10) UNSIGNED NOT NULL,
`imageurl` varchar(255) NOT NULL,
`show` tinyint(1) NOT NULL DEFAULT '1'
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
--
-- 转存表中的数据 `fz_welcome`
--
INSERT INTO `fz_welcome` (`id`, `imageurl`, `show`) VALUES
(35, '/UpLoads/welcome/images/2016-05-10/5730befdbf790.jpg', 1),
(36, '/UpLoads/welcome/images/2016-05-10/5730bf15ca8cf.jpg', 1),
(37, '/UpLoads/welcome/images/2016-05-10/5730bf3d625df.jpg', 1),
(38, '/UpLoads/welcome/images/2016-05-10/5730bf4ba287f.jpg', 1),
(40, '/UpLoads/welcome/images/2016-05-10/5730bf8944e8b.jpg', 1),
(41, '/UpLoads/welcome/images/2016-05-10/573169d431643.jpg', 1);
--
-- Indexes for dumped tables
--
--
-- Indexes for table `fz_access`
--
ALTER TABLE `fz_access`
ADD KEY `groupId` (`role_id`),
ADD KEY `nodeId` (`node_id`);
--
-- Indexes for table `fz_cart`
--
ALTER TABLE `fz_cart`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `fz_node`
--
ALTER TABLE `fz_node`
ADD PRIMARY KEY (`id`),
ADD KEY `level` (`level`),
ADD KEY `pid` (`pid`),
ADD KEY `status` (`status`),
ADD KEY `name` (`name`);
--
-- Indexes for table `fz_pattr`
--
ALTER TABLE `fz_pattr`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `fz_product`
--
ALTER TABLE `fz_product`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `fz_ptype`
--
ALTER TABLE `fz_ptype`
ADD PRIMARY KEY (`id`),
ADD KEY `pid` (`pid`);
--
-- Indexes for table `fz_role`
--
ALTER TABLE `fz_role`
ADD PRIMARY KEY (`id`),
ADD KEY `pid` (`pid`),
ADD KEY `status` (`status`);
--
-- Indexes for table `fz_role_user`
--
ALTER TABLE `fz_role_user`
ADD KEY `group_id` (`role_id`),
ADD KEY `user_id` (`user_id`);
--
-- Indexes for table `fz_shop`
--
ALTER TABLE `fz_shop`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `fz_shop_home`
--
ALTER TABLE `fz_shop_home`
ADD PRIMARY KEY (`shop_id`);
--
-- Indexes for table `fz_user`
--
ALTER TABLE `fz_user`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `fz_user_copy`
--
ALTER TABLE `fz_user_copy`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `fz_welcome`
--
ALTER TABLE `fz_welcome`
ADD PRIMARY KEY (`id`);
--
-- 在导出的表使用AUTO_INCREMENT
--
--
-- 使用表AUTO_INCREMENT `fz_cart`
--
ALTER TABLE `fz_cart`
MODIFY `id` int(10) UNSIGNED NOT NULL AUTO_INCREMENT;
--
-- 使用表AUTO_INCREMENT `fz_node`
--
ALTER TABLE `fz_node`
MODIFY `id` smallint(6) UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=126;
--
-- 使用表AUTO_INCREMENT `fz_pattr`
--
ALTER TABLE `fz_pattr`
MODIFY `id` int(10) UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=52;
--
-- 使用表AUTO_INCREMENT `fz_product`
--
ALTER TABLE `fz_product`
MODIFY `id` int(11) UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=65;
--
-- 使用表AUTO_INCREMENT `fz_ptype`
--
ALTER TABLE `fz_ptype`
MODIFY `id` int(11) UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=32;
--
-- 使用表AUTO_INCREMENT `fz_role`
--
ALTER TABLE `fz_role`
MODIFY `id` smallint(6) UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=22;
--
-- 使用表AUTO_INCREMENT `fz_shop`
--
ALTER TABLE `fz_shop`
MODIFY `id` int(11) UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=31;
--
-- 使用表AUTO_INCREMENT `fz_user`
--
ALTER TABLE `fz_user`
MODIFY `id` int(11) UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=120;
--
-- 使用表AUTO_INCREMENT `fz_user_copy`
--
ALTER TABLE `fz_user_copy`
MODIFY `id` int(11) UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=120;
--
-- 使用表AUTO_INCREMENT `fz_welcome`
--
ALTER TABLE `fz_welcome`
MODIFY `id` int(10) UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=42;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
<file_sep>/Apps/Admin/Controller/RoleController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
class RoleController extends AuthController {
/**
* 显示角色管理页面
*/
public function index() {
$this->role = M ( 'role' )->select ();
$this->display ();
}
/**
* 添加角色
*/
public function add() {
if (! IS_POST)
$this->error ( "页面不存在!" );
if ($_POST ['role_name'] == null || $_POST ['role_status'] == null || $_POST ['role_remark'] == null) {
$this->error ( "传入参数错误!" );
}
$role = array (
'name' => $_POST ['role_name'],
'status' => $_POST ['role_status'],
'remark' => $_POST ['role_remark']
);
if (M ( 'role' )->add ( $role )) {
$this->redirect ( "/Admin/Role" );
} else {
$this->error ( "添加角色错误!" );
}
}
/**
* 删除角色
*/
public function del() {
if (! IS_POST) $this->error ( "页面不存在!" );
$role = array (
'id' => $_POST ['id'],
'name' => $_POST ['name'],
'status' => $_POST ['status'],
'remark' => $_POST ['remark']
);
M ( 'role' )->where (array($role ))->delete ();
}
/**
* 改变角色状态
*/
public function status() {
if (! IS_POST) $this->error ( "页面不存在!" );
$role = array (
'id' => $_POST ['id'],
'name' => $_POST ['name'],
'status' => $_POST ['status'],
'remark' => $_POST ['remark']
);
M ( 'role' )->where($role)->save(array('status'=>!$role['status']));
}
}<file_sep>/Apps/API/Controller/WelcomeController.class.php
<?php
namespace API\Controller;
use Think\Controller\RestController;
class WelcomeController extends RestController {
protected $allowMethod = array('get','post','put','delete'); // REST允许的请求类型列表
protected $allowType = array('json'); // REST允许请求的资源类型列表
public function index() {
header ( 'Cache-Control:max-age=0' );
header ( 'Content-Type:text/html;charset=utf-8 ', true );
header ( 'Last-Modified: ' . gmdate ( 'D, d M Y H:i:s', time () ) . ' GMT', true );
switch ($this->_method) {
case 'get' : // get请求处理代码
if(!$_GET){
$data = M('welcome')->select();
}else{
$data = M('welcome')->where(array('id'=>$_GET['id']))->find();
}
$this->response($data,'json');
break;
case 'put' : // put请求处理代码
break;
case 'post' : // post请求处理代码
break;
case 'delete' : // delete请求处理代码
break;
}
}
}<file_sep>/Apps/Common/Model/RolePathModel.class.php
<?php
namespace Common\Model;
use Think\Model\RelationModel;
class RolePathModel extends RelationModel{
//定义主表
protected $tableName = 'role';
//定义关联关系
protected $_link = array(
'node'=>array(
'mapping_type' => self::MANY_TO_MANY,
'class_name' => 'node',
'foreign_key'=>'role_id',
'relation_key'=>'node_id',
'relation_table'=>'fz_access',
'mapping_fields'=>'id,name,pid'
)
);
}<file_sep>/Apps/Admin/Controller/LoginController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
class LoginController extends Controller {
public function index() {
$this->display ();
}
public function login(){
if(!IS_POST) $this->error("页面不存在!");
$db = M('shop');
$data = $db->where(array('shopname'=>$_POST['shopname'],'loginword'=>md5($_POST['password'])))->find();
if($data==null) $this->error("用户名密码错误!");
//添加记录登陆信息代码;
import("Cls.User",APP_PATH);
$log = \User::insertLog('shop_log',$data['id'],'shop_id');
if(!$log) $this->error("更新登陆信息失败!");
//session
session(C('USER_AUTH_KEY'),$data['id']);
session('shopname',$data['shopname']);
session('loginIP',$log['loginIP']);
session('logtime',$log['logintime']);
//如果是超级管理员,不需要认证
if($data['shopname']==C('RBAC_SUPERADMIN')){
session(C('ADMIN_AUTH_KEY'),true);
}
//RBAC
\Org\Util\Rbac::saveAccessList();
// dump($_SESSION);
// die();
//其它需要的操作
$this->redirect("/Admin");//跳转到主页
}
public function logout(){
session_unset();
session_destroy();
$this->redirect("/Admin/Login");
}
}<file_sep>/index.php
<?php
// define('BIND_MODULE','Admin');
// define('BUILD_CONTROLLER_LIST','Admin');
// define('BUILD_MODEL_LIST','Home','API','Admin');
define ( 'APP_DEBUG', TRUE ); // 开启调试模式 建议开发阶段开启 部署阶段注释或者设为false
define ( 'APP_PATH', './Apps/' ); // 定义应用目录
define ( 'RUNTIME_PATH', './Runtime/' ); // 定义运行时目录
require './ThinkPHP/ThinkPHP.php'; // 引入ThinkPHP入口文件
?>
<file_sep>/Apps/Admin/Controller/HomeShopController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
class HomeShopController extends AuthController {
public function index(){
$shops = D('ShopAll')->field('loginword,token,describe,regIP,describe,countshop,countmoney,level,lock,type',true)
->where(array('type'=>1))
->relation('show')
->select();
$this->shops=$shops;
// dump($shops);
$this->display();
}
public function add(){
if(!IS_POST) die();
M('shop_home')->add(array('shop_id'=>I('shop_id'),'show'=>1));
}
public function del(){
if(!IS_POST) die();
M('shop_home')->where(array('shop_id'=>I('shop_id')))->delete();
}
}<file_sep>/Apps/Admin/Public/js/admin-node.js
$(document).ready(function() {
$('#add').click(function() {
if ($('#node_name').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#node_name').focus();
return false;
}
if ($('#node_remark').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#node_remark').focus();
return false;
}
$('#addfrom').submit();
});
});
function closeAddDlg(s1, s2, s3, s4, s5, s6) {
$('.node-dlg-add').fadeOut(100);
$('.node-mask').fadeOut(100);
}
function addDlg(s1, s2, s3) {
$('.node-dlg-add').fadeIn(100);
$('.node-mask').fadeIn(100);
// 设置初始值
document.getElementById("parent_name1").value = s1;
document.getElementById("node_pid").value = s2;
document.getElementById("node_level").value = s3;
}
function closeEditDlg(s1, s2, s3, s4, s5, s6) {
$('.node-dlg-edit').fadeOut(100);
$('.node-mask').fadeOut(100);
}
function editDlg(s1, s2, s3, s4, s5, s6,s7) {
$('.node-dlg-edit').fadeIn(100);
$('.node-mask').fadeIn(100);
// 设置初始值
document.getElementById("edit_id").value = s1;
document.getElementById("edit_name").value = s2;
document.getElementById("edit_remark").value = s3;
document.getElementById("edit_status").value = s4;
document.getElementById("edit_pid").value = s5;
document.getElementById("edit_level").value = s6;
document.getElementById("parent_name2").value = s7;
}
function delDlg(s1, s2, s3, s4, s5, s6) {
$.ajax({
url : delurl,
type : 'post',
data : "id=" + s1 + "&name=" + s2 + "&remark=" + s3 + "&status=" + s4
+ "&pid=" + s5 + "&level=" + s6,
success : function() {
parent.location.reload();
window.location.reload();
},
dataType : 'html'
});
}<file_sep>/Apps/Admin/Controller/AuthController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
/**
* Rbac认证基类,所有需要认证的控制器必须继续此类
* @author Administrator
*
*/
class AuthController extends Controller {
public function _initialize() {
$this->username=$_SESSION ['shopname'];
//不需要认证的控制器
$notModel = in_array(CONTROLLER_NAME,explode(',',C('NOT_AUTH_CONTROLLER')));
// if($notModel) {
// $this->display ( "Auth/index" );
// return ;
// }
//不需要认证的方法
//没有登陆跳转到登陆界面
if (! isset ( $_SESSION [C ( 'USER_AUTH_KEY' )] )&&!$notModel) {
$this->redirect ( "/Admin/Login" );
}
//没有访问权限返回原来目录
if(C('USER_AUTH_ON')&&!$notModel){
\Org\Util\Rbac::AccessDecision()||$this->error("没有访问权限");
}
//找出登陆用户可以访问的所有路径确定哪些菜单可以显示
$id = $_SESSION [C ( 'USER_AUTH_KEY' )] ;
$user = M('role_user')->where(array('user_id'=>$id))->find();
$data=D('RolePath')->relation(true)->field('id')->where(array('id'=>$user['role_id']))->find();
$this->paths=path_merge($data['node']);
//如果是超级用户,显示所有菜单
$this->super=$_SESSION [C ( 'ADMIN_AUTH_KEY' )]!=null;
$this->display ( "Auth/index" );
}
}<file_sep>/Apps/Admin/Conf/config.php
<?php
return array (
// '配置项'=>'配置值'
//---RBAC配置-----/
// 超级管理员名称
'RBAC_SUPERADMIN' => 'flyzebra',
// 超级管理员识别
'ADMIN_AUTH_KEY' => 'superman',
// 是否开启验证
'USER_AUTH_ON' => true,
// 验证类型(1:登陆验证,2:实时验证)
'USER_AUTH_TYPE' => 2,
// 用户认证识别号
'USER_AUTH_KEY' => 'uid',
// 无需认证的控制器
'NOT_AUTH_CONTROLLER' => 'Register,Index',
// 无需认证的动作方法
// 'NOT_AUTH_ACTION' => 'login,logout,register',
// 角色表名称
'RBAC_ROLE_TABLE' => 'fz_role',
// 角色与用户的中间表名称
'RBAC_USER_TABLE' => 'fz_role_user',
// 权限表名称
'RBAC_ACCESS_TABLE' => 'fz_access',
// 节点表名称
'RBAC_NODE_TABLE' => 'fz_node',
//---RBAC配置-----/
//配置__PUBLIC__
'TMPL_PARSE_STRING'=>array(
// 站点资源文件目录
'__PUBLIC__' => __ROOT__.'/Apps/Admin/Public',
),
//默认错误跳转对应的模板文件
'TMPL_ACTION_ERROR' => 'JumpHtml:error',//默认成功跳转对应的模板文件
'TMPL_ACTION_SUCCESS' => 'JumpHtml:success',
);<file_sep>/Apps/Admin/Controller/TypeController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
class TypeController extends AuthController {
/**
* 商品分类管理
*/
public function index() {
$this->ptype=M('ptype')->select();
$this->display();
}
/**
* 添加商品分类
*/
public function add(){
if(!IS_POST) $this->error("页面不存在!");
if($_POST['pid']==null||$_POST['ptype_name']==null||$_POST['order']==null){
$this->error("传入参数错误!");
}
$ptypDao = M('ptype');
if($ptypDao->add($_POST)){
$this->redirect("/Admin/Type");
}else{
$this->error($ptypDao->getDbError());
}
}
/**
* 删除商品分类
*/
public function del(){
if (! IS_POST) $this->error("页面不存在!");
$ptypDao = M('ptype');
if($ptypDao->where(array($_POST))->delete()){
$this->redirect("/Admin/Type");
}else{
$this->error($ptypDao->getError());
}
}
}<file_sep>/Apps/API/Controller/IndexController.class.php
<?php
namespace API\Controller;
use Think\Controller;
class IndexController extends Controller {
public function index() {
header ( 'Cache-Control:max-age=600' );
header ( 'Content-Type:text/html;charset=utf-8 ', true );
header ( 'Last-Modified: ' . gmdate ( 'D, d M Y H:i:s', time () ) . ' GMT', true );
echo 'Content-Type:text/html;charset=utf-8 <br>';
echo 'Cache-Control, max-age=600<br>';
echo 'Last-Modified: ' . gmdate ( 'D, d M Y H:i:s', time () ) . ' GMT<br>';
echo __ACTION__ . "<hr>";
}
}<file_sep>/Apps/Admin/Controller/ProductController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
class ProductController extends AuthController {
/**
* 显示添加商品页面
*/
public function index(){
$this->pattr=M('pattr')->select();
$this->ptype=M('ptype')->select();
$this->shopname = $_SESSION['shopname'];
$this->shop_id = $_SESSION[C('USER_AUTH_KEY')];
$this->display();
}
/**
* 添加商品
*/
public function add(){
if(!IS_POST) $this->error ("页面不存在!");
$product = $_POST;
$upload = new \Think\Upload (); // 实例化上传类
$upload->maxSize = 3145728; // 设置附件上传大小
$upload->exts = array ( 'jpg','png'); // 设置附件上传类型
$upload->rootPath = './UpLoads/';
$upload->savePath = '/product/images/'; // 设置附件上传目录
// 更新product(产品)表
//检测数据完整性
$product['shopcount'] = 0;
$pattr_ids = $product['pattr_id'];
unset($product['pattr_id']);
$product['addtime'] = time();
$proinfoDao = M('product');
$product_id = $proinfoDao->add($product);
if(!$product_id) $this->error ("添加商品失败!");
//更新pattr(产品属性)表
$product_pattrDao = M('product_pattr');
foreach ( $pattr_ids as $pattr_id ) {
if(!$product_pattrDao->add(array('product_id'=>$product_id,'pattr_id'=>$pattr_id))){
$this->error("添加商品属性失败!");
}
}
// 接受上传的图像文件
$info = $upload->upload ();
if (! $info) { // 上传错误提示错误信息
$this->error ("上传图像文件失败!");
} else {
//更新product_imgurl(产品图像)表
$product_imgurlDao = M('product_imgurl');
foreach ( $info as $file ) {
$imgurl = '/UpLoads'.$file ['savepath'] . $file ['savename'];
//处理图像.....图像是上传后处理成统一格式还是显示的时候处理
import ( "Fly.MyImage", "." );
\MyImage::thumb ( "." . $imgurl, 400, 400);
if(!$product_imgurlDao->add(array('product_id'=>$product_id,'imgurl'=>$imgurl))){
$this->error("添加图像文件失败!");
}
}
}
$this->success("添加商品成功");
}
public function find(){
$this->display('Product/find');
}
}<file_sep>/Apps/Admin/Public/js/admin-register.js
$(document).ready(function() {
$('#register').click(function() {
if ($('#shopname').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#shopname').focus();
return false;
}
if ($('#peoplename').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#peoplename').focus();
return false;
}
if ($('#loginword').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#loginword').focus();
return false;
}
if ($('#loginword2').val() != $('#loginword').val()) {
$('#message').html("<font color='red'><b>两次输入密码不一致!</b></font>");
$('#loginword2').focus();
return false;
}
if ($('#peoplephone').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#peoplephone').focus();
return false;
}
if ($('#shopphone').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#shopphone').focus();
return false;
}
if ($('#address').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#address').focus();
return false;
}
if ($('#proimg1').val() == "") {
$('#proimg1').focus();
return false;
}
// if ($('#proimg2').val() == "") {
// $('#proimg2').focus();
// return false;
// }
// if ($('#proimg3').val() == "") {
// $('#proimg3').focus();
// return false;
// }
if ($('#describe').val() == "") {
$('#describe').focus();
return false;
}
var loginword = hex_md5(document.getElementById("loginword").value);
$.ajax({
url:ajaxurl,
type:"post",
data:"shopname=" + $("#shopname").val(),
dataType:'html',
success: function(result) {
if (result.length > 2) {
$('#message').html("<font color='red'><b>"+result+"</b></font>");
return false;
} else {
$('#message').html("<font color='blue'><b>注册请求已提交!</b></font>");
document.getElementById("loginword").value = loginword;
$('#registerFrom').submit();
}
}
});
});
});
<file_sep>/Runtime/Cache/Admin/515eaa81c30b431e825eb4fdfdd05f1b.php
<?php if (!defined('THINK_PATH')) exit();?>
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>宇翔科技后台管理</title>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/jquery-1.9.0.min.js"></script>
<script type="text/javascript"> var delurl= '<?php echo U("/Admin/Role/del");?>'</script>
<script type="text/javascript"> var statusurl= '<?php echo U("/Admin/Role/status");?>'</script>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/admin-role.js"></script>
</head>
<body>
<div class="crumb-wrap">
<span class="icon-font">  <a href="<?php echo U('/Admin');?>">首页</a></span>
<span class="crumb-step">> <a href="#">添加角色</a></span>
</div>
<div class="main-wrap">
<div class="result-wrap">
<table class="admin-tab" width="900px">
<tbody align="right">
<tr style="background:#e8e8e8">
<th width=25%>角色名称</th>
<th width=25%>角色描述</th>
<th width=25%>使用状态</th>
<th width=25%>执行操作</th>
</tr>
<?php if(is_array($role)): foreach($role as $key=>$v): ?><tr>
<th><?php echo ($v["name"]); ?></th>
<th><?php echo ($v["remark"]); ?></th>
<th><?php if($v["status"]): ?>启用<?php else: ?>停用<?php endif; ?></th>
<th>
<a href="javascript:poststatus('<?php echo ($v["id"]); ?>','<?php echo ($v["name"]); ?>','<?php echo ($v["pid"]); ?>','<?php echo ($v["status"]); ?>','<?php echo ($v["remark"]); ?>');">
<i class="icon-font">
<?php if($v["status"]): ?>停用<?php else: ?>启用<?php endif; ?>
</i>
</a> 
<a href="javascript:postdel('<?php echo ($v["id"]); ?>','<?php echo ($v["name"]); ?>','<?php echo ($v["pid"]); ?>','<?php echo ($v["status"]); ?>','<?php echo ($v["remark"]); ?>');">
<i class="icon-font">删除</i>
</a>
</th>
</tr><?php endforeach; endif; ?>
</tbody>
</table>
<br>
<form name="addFrom" id="addFrom" accept-charset="utf-8" action="<?php echo U('/Admin/Role/add');?>" method="post">
<table class="admin-tab" width="900px">
<tbody>
<tr>
<th style="background:#e8e8e8" colspan="2">添加角色</th>
</tr>
<tr>
<th><span class="require-red">*</span>角色名称:</th>
<td>
<input class="common-text" type="text" id="role_name" name="role_name" size="40" value="">
</td>
</tr>
<tr>
<th><span class="require-red">*</span>角色描述:</th>
<td>
<input class="common-text" type="text" id="role_remark" name="role_remark" size="40" value="">
</td>
</tr>
<tr>
<th><span class="require-red">*</span>是否启用:</th>
<td>
<input class="common-text" id="role_status" name="role_status" value="1" type="radio" checked = "checked">开启
<input class="common-text" id="role_status" name="role_status" value="0" type="radio">关闭
</td>
</tr>
<tr>
<th></th>
<td>
<input type="submit"class="btn btn-primary btn6 mr10" value="添加" id="add">
<input type="reset" class="btn btn-primary btn6 mr10" value="重置">
</td>
</tr>
</tbody>
</table>
</form>
</div>
</div>
</body>
</html><file_sep>/Apps/Admin/Controller/IndexController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
class IndexController extends AuthController {
public function index(){
for($i=0;$i<10;$i++){
echo "00".$i."<i class=\"icon-font\">฀".$i.";</i>";
}
for($i=10;$i<70;$i++){
echo "0".$i."<i class=\"icon-font\">à".$i.";</i>";
}
}
}<file_sep>/Runtime/Cache/Home/400c995c330f1f6ecac6b9102849eb7e.php
<?php if (!defined('THINK_PATH')) exit();?><!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>宇翔电脑科技有限公司</title>
<link rel="stylesheet" href="/myweb/xinyi/Public/css/reset.css"/>
<link rel="stylesheet" href="/myweb/xinyi/Public/css/font-awesome.min.css"/>
<link rel="stylesheet" href="/myweb/xinyi/Public/css/style.css"/>
</head>
<body>
<!--scroll-head-->
<div class="scroll-head"></div>
<!--top-->
<div class="top-wrapper">
<div class="top-info">
<div class="top-left">
<div data-toggle="arrowdown" id="arrow1" class="user-name">
<a href="#">站长素材</a>
<span class="down-icon"></span>
</div>
<div data-toggle="arrowdown" id="arrow2" class="msg-info">
<i class="fa fa-envelope fa-gray"></i>
<a href="#">消息</a>
<span class="down-icon"></span>
</div>
<!--hidden-box-->
<div data-toggle="hidden-box" id="nav-box1" class="user-box">
<img class="my-head" src="/myweb/xinyi/Public/img/user-head.jpg" />
<div class="my-grow">
<h1><a href="#">账号管理</a> | <a href="#">退出</a></h1>
<p>
<h2><a href="#">查看我会员特权</a></h2>
<a href="#">我的成长</a>
</p>
</div>
<p style="height: 10px; clear: both;"> </p>
<div class="my-card">
<div class="cards-info">
<ul>
<li><img src="/myweb/xinyi/Public/img/icon.png" /></li>
<li><img src="/myweb/xinyi/Public/img/icon.png" /></li>
</ul>
</div>
<div class="toggle-box">
<div class="toggle">
<i style="vertical-align: top" class="fa fa-angle-left arrow-left"></i>
<i style="vertical-align: top" class="fa fa-angle-right arrow-right"></i>
</div>
</div>
</div>
</div>
<div data-toggle="hidden-box" id="nav-box2" class="msg-box">
<h1>未读新消息<a href="#" class="fa fa-pencil-square-o pencil"></a></h1>
<div class="read-info">
<h2><span class="orange"> |</span> 你的书架 <span style="font-weight: lighter">收到了<span class="orange">1</span>本书</span><span class="fa fa-times close-msg"></span></h2>
<img src="/myweb/xinyi/Public/img/book-1.png" />
<div style="float: right">
<p>
全中国最穷的小伙子发财日记<br/>
掏彩票公共账号
</p>
<h3><a href="#">去看看</a></h3>
</div>
</div>
<div class="msg-setting">
<a href="#" class="fa fa-cog"></a>
<a href="#" class="fa fa-pencil-square-o"></a>
<a style="margin-left: 70px" href="#">买家消息 | </a>
<a href="#">卖家消息</a>
</div>
</div>
</div>
<!--top-right-->
<div class="top-right">
<div data-toggle="arrowdown" id="arrow3" class="user-name">
<a href="#">未登陆</a>
<span class="down-icon"></span>
</div>
<div data-toggle="arrowdown" id="arrow4" class="user-name">
<i class="fa fa-shopping-cart fa-orange"></i>
<a href="#">购物车</a>
<span class="down-icon"></span>
</div>
<div data-toggle="arrowdown" id="arrow5" class="user-name">
<i class="fa fa-star fa-gray"></i>
<a href="#">收藏夹</a>
<span class="down-icon"></span>
</div>
<a class="a-float-left" href="#">商品分类</a>
<span class="vertical-line">|</span>
<div data-toggle="arrowdown" id="arrow6" class="user-name">
<a href="#">卖家中心</a>
<span class="down-icon"></span>
</div>
<a class="a-float-left" href="#">联系客户</a>
<div data-toggle="arrowdown" id="arrow7" class="user-name">
<i class="fa fa-list-ul fa-orange"></i>
<a href="#">网站导航</a>
<span class="down-icon"></span>
</div>
<!--hidden-box-->
<div data-toggle="hidden-box" id="nav-box3" class="my-taobao-box">
<ul>
<li>已买到的宝贝</li>
<li>新欢</li>
<li>我的足迹</li>
<li>我的上新</li>
<li>我的优惠</li>
</ul>
</div>
<div data-toggle="hidden-box" id="nav-box4" class="shopping-box">
<span>您购物车里还没有任何宝贝。</span><a class="check-shopp" href="#">查看我的购物车</a>
</div>
<div data-toggle="hidden-box" id="nav-box5" class="get-box">
<ul>
<li>收藏的宝贝</li>
<li>收藏的店铺</li>
</ul>
</div>
<div data-toggle="hidden-box" id="nav-box6" class="center-box">
<ul>
<li>已卖出的宝贝</li>
<li>出售中的宝贝</li>
<li>卖家服务市场</li>
<li>卖家培训中心</li>
</ul>
</div>
<div data-toggle="hidden-box" id="nav-box7" class="nav-box">
<div class="sub-nav top-sub-nav">
<!--<input type="button" class="more-btn" value="更多"/>-->
<!--sub-nav-cell-->
<div class="sub-nav-cell right-shadow">
<h3 class="orange">主题市场</h3>
<ul class="sub-nav-box">
<li><a href="#">爱美女人</a></li>
<li><a href="#">品质男人</a></li>
<li><a href="#">淘宝二手</a></li>
<li><a href="#">全球购</a></li>
<li><a href="#">品牌街</a></li>
<li><a href="#">汽车生活</a></li>
<li><a href="#">花嫁新娘</a></li>
<li><a href="#">孕婴童</a></li>
<li><a href="#">同城便民</a></li>
<li><a href="#">爱么装</a></li>
<li><a href="#">运动户外</a></li>
<li><a href="#">家用电器</a></li>
<li><a href="#">狠爱</a></li>
<li><a href="#">网络游戏</a></li>
<li><a href="#">潮流设计</a></li>
<li><a href="#">淘宝星愿</a></li>
</ul>
</div>
<div class="sub-nav-cell right-shadow">
<h3 class="green-sp">特色购物</h3>
<ul class="sub-nav-box sp">
<li><a href="#">爱美女人</a></li>
<li><a href="#">品质男人</a></li>
<li><a href="#">淘宝二手</a></li>
<li><a href="#">全球购</a></li>
<li><a href="#">品牌街</a></li>
<li><a href="#">汽车生活</a></li>
<li><a href="#">花嫁新娘</a></li>
<li><a href="#">孕婴童</a></li>
<li><a href="#">同城便民</a></li>
<li><a href="#">爱么装</a></li>
<li><a href="#">运动户外</a></li>
<li><a href="#">家用电器</a></li>
<li><a href="#">狠爱</a></li>
<li><a href="#">网络游戏</a></li>
<li><a href="#">潮流设计</a></li>
<li><a href="#">淘宝星愿</a></li>
</ul>
</div>
<div class="sub-nav-cell right-shadow">
<h3 class="red-now">当前热点</h3>
<ul class="sub-nav-box now">
<li><a href="#">爱美女人</a></li>
<li><a href="#">品质男人</a></li>
<li><a href="#">淘宝二手</a></li>
<li><a href="#">全球购</a></li>
<li><a href="#">品牌街</a></li>
<li><a href="#">汽车生活</a></li>
<li><a href="#">花嫁新娘</a></li>
<li><a href="#">孕婴童</a></li>
<li><a href="#">同城便民</a></li>
<li><a href="#">爱么装</a></li>
<li><a href="#">运动户外</a></li>
<li><a href="#">家用电器</a></li>
<li><a href="#">狠爱</a></li>
<li><a href="#">网络游戏</a></li>
<li><a href="#">潮流设计</a></li>
<li><a href="#">淘宝星愿</a></li>
</ul>
</div>
<div class="sub-nav-cell">
<h3 class="blue-more">更多精彩</h3>
<ul class="sub-nav-box more">
<li><a href="#">爱美女人</a></li>
<li><a href="#">品质男人</a></li>
<li><a href="#">淘宝二手</a></li>
<li><a href="#">全球购</a></li>
<li><a href="#">品牌街</a></li>
<li><a href="#">汽车生活</a></li>
<li><a href="#">花嫁新娘</a></li>
<li><a href="#">孕婴童</a></li>
<li><a href="#">同城便民</a></li>
<li><a href="#">爱么装</a></li>
<li><a href="#">运动户外</a></li>
<li><a href="#">家用电器</a></li>
<li><a href="#">狠爱</a></li>
<li><a href="#">网络游戏</a></li>
<li><a href="#">潮流设计</a></li>
<li><a href="#">淘宝星愿</a></li>
</ul>
</div>
</div>
</div>
</div>
</div>
<!--scroll-top-->
<div class="scroll-search">
<div class="search-litter">
<img class="scroll-logo" src="/myweb/xinyi/Public/img/logo.png" />
<!--scroll-search-->
<div class="search-wrapper-scroll">
<div class="search-box-scroll">
<div data-toggle="arrowdown" id="arrow9" class="search-toggle-scroll">
宝贝<span class="down-icon"></span>
</div>
<input class="search-in-scroll" type="text" placeholder=" 搜‘健康椅’试试,办公好选择" />
<input type="button" class="search-but-scroll" value="搜索">
<div data-toggle="hidden-box" id="nav-box9" class="search-toggle-box-scroll">店铺</div>
</div>
</div>
</div>
</div>
</div>
<!--top-main-->
<div class="top-main">
<img src="/myweb/xinyi/Public/img/logo.gif" />
<div class="search-wrapper">
<div class="search-box">
<div data-toggle="arrowdown" id="arrow8" class="search-toggle">
宝贝<span class="down-icon"></span>
</div>
<input class="search-in" type="text" placeholder=" 输入商家或商品名称">
<input type="button" class="search-but" value="搜索">
<div data-toggle="hidden-box" id="nav-box8" class="search-toggle-box">店铺</div>
</div>
<a class="a-float-left hight-search" href="#">高级<br/>搜索</a>
<!--other-search-->
</div>
<!--two-code-->
</div>
<!--content-top-->
<div class="content-top">
<!--sidebar-->
<div class="sidebar">
<h3>商品服务分类</h3>
<!--sidebar-info-->
<div class="sidebar-info">
<ul class="side-li">
<li class="s_1"><h3>服装内衣<span class="fa fa-angle-right fa-loc"></span></h3></li>
</ul>
<!--hidden-li-box-->
<ul class="hiden-box">
<li data-hidden="li" id="hiden-1">
<div class="hidden-title">
<a href="#">淘宝女人</a><span class="right-box"><i class="fa fa-angle-right"></i></span>
<a href="#">最in女装</a><span class="right-box"><i class="fa fa-angle-right"></i></span>
<a href="#">淘宝男人</a><span class="right-box"><i class="fa fa-angle-right"></i></span>
<a href="#">女人搭配</a><span class="right-box"><i class="fa fa-angle-right"></i></span>
</div>
<div class="sub-nav-right">
<div class="cell-box">
<h1>印记女装</h1>
<div class="a-box">
<a href="#">连衣裙</a><span>|</span>
<a href="#">裤子</a><span>|</span>
<a href="#">小西装</a><span>|</span>
<a href="#">长袖村上</a><span>|</span>
<a href="#" class="orange">春季外套</a>
<a href="#">郑志山</a><span>|</span>
<a href="#">毛衣</a><span>|</span>
<a href="#">长袖T恤</a><span>|</span>
<a href="#">唯一</a><span>|</span>
<a href="#">雪绒钱</a><span>|</span>
<a href="#">大地亏</a>
<a href="#">半身全</a><span>|</span>
<a href="#">风衣</a><span>|</span>
<a href="#">类似雪纺</a><span>|</span>
<a href="#">毛衣外套</a><span>|</span>
<a href="#">棒球服</a>
<a href="#">牛仔装</a><span>|</span>
<a href="#">礼服</a><span>|</span>
<a href="#">婚纱</a><span>|</span>
<a href="#">大妈</a><span>|</span>
<a href="#">Zara</a><span>|</span>
<a href="#">挨个</a>
</div>
</div>
</div>
<div class="sub-nav-left">
<div class="cell-box">
<h1>印记女装</h1>
<div class="a-box">
<a href="#">连衣裙</a><span>|</span>
<a href="#">裤子</a><span>|</span>
<a href="#">小西装</a><span>|</span>
<a href="#">长袖村上</a><span>|</span>
<a href="#" class="orange">春季外套</a>
<a href="#">郑志山</a><span>|</span>
<a href="#">毛衣</a><span>|</span>
<a href="#">长袖T恤</a><span>|</span>
<a href="#">唯一</a><span>|</span>
<a href="#">雪绒钱</a><span>|</span>
<a href="#">大地亏</a>
<a href="#">半身全</a><span>|</span>
<a href="#">风衣</a><span>|</span>
<a href="#">类似雪纺</a><span>|</span>
<a href="#">毛衣外套</a><span>|</span>
<a href="#">棒球服</a>
<a href="#">牛仔装</a><span>|</span>
<a href="#">礼服</a><span>|</span>
<a href="#">婚纱</a><span>|</span>
<a href="#">大妈</a><span>|</span>
<a href="#">Zara</a><span>|</span>
<a href="#">挨个</a>
</div>
</div>
</div>
</li>
</ul>
</div>
</div>
<!--right-con-->
<div class="right-con">
<div class="nav">
<a id="spe-a1" href="#">天猫</a>
<a id="spe-a2" href="#">聚划算</a>
<a id="spe-a3" href="#">二手</a>
<span class="line-a">|</span>
<a href="#">拍卖</a>
<a href="#">一淘</a>
<a href="#">电器城</a>
<a href="#">Hitao粉妆</a>
<a href="#">旅行</a>
<a href="#">云手机</a>
<a href="#">特色中国</a>
<img style="cursor: pointer" src="/myweb/xinyi/Public/img/ad.gif" />
<span class="keep-a" href="#"><a href="#">消费者保障</a></span>
</div>
<!--show-box-->
<div class="show-box">
<!--content-->
<div class="content">
<ul class="imgBox">
<?php if(is_array($shops)): foreach($shops as $key=>$v): ?><li><a href="#"><img style="width:720px; height:530px" src="/myweb/xinyi<?php echo ($v["imgurl"]); ?>"></a></li><?php endforeach; endif; ?>
</ul>
<div class="currentNum">
<span class="imgNum mark-color"></span>
<span class="imgNum"></span>
<span class="imgNum"></span>
<span class="imgNum"></span>
<span class="imgNum"></span>
</div>
<div class="control to-left"><i class="fa fa-angle-left"></i></div>
<div class="control to-right"><i class="fa fa-angle-right"></i></div>
</div>
<!--content-down-->
</div>
<!--right-sidbar-->
<div class="right-sidebar">
<div class="info-box">
<ul class="tab-nav">
<li id="li-1" class="li-nav li-nav-hover li-border">公告</li>
<li id="li-2" class="li-nav">规则</li>
<li id="li-3" class="li-nav">论坛</li>
<li id="li-4" class="li-nav">安全</li>
<li style="border-right: none" id="li-5" class="li-nav">公益</li>
</ul>
<div id="box-1" style="display: block" class="hiddenBox">
<a href="#">淘宝2014:开放多样性</a>
<a href="#">舌尖中国二季 天猫首尝</a>
<a href="#">阿里通信自建计费系统</a>
<a href="#">来往首届419宇宙节</a>
</div>
<div id="box-2" class="hiddenBox">
<a href="#">[重要] 淘点点规范</a>
<a href="#">[重要] 虚假交易新规 </a>
<a href="#">[重要] 新增认证规则</a>
<a href="#">[重要] 购买刀具实名</a>
</div>
<div id="box-3" class="hiddenBox">
<a href="#">[优化] 称谓滥用将整改</a>
<a href="#">[热点] 如何赢取新商机</a>
<a href="#">[话题] 同城交易避处罚</a>
<a href="#">[聚焦] 新消保法全解析</a>
</div>
<div id="box-4" class="hiddenBox">
<a href="#">个人重要信息要管牢!</a>
<a href="#">卖家防范红包欺诈提醒</a>
<a href="#">更换收货地址的陷阱!</a>
<a href="#">注意骗子的技术升级了</a>
</div>
<div id="box-5" class="hiddenBox">
<a href="#">[优化] 称谓滥用将整改</a>
<a href="#">[热点] 如何赢取新商机</a>
<a href="#">[话题] 同城交易避处罚</a>
<a href="#">[聚焦] 新消保法全解析</a>
</div>
</div>
<!--user-info-->
<div class="user-info">
<div class="gold-top">
<img width="62px" height="62px" src="/myweb/xinyi/Public/img/user-head.jpg" />
<div class="inner-user">
<h3>Hi 天之狼2011</h3>
<a class="get-gold" href="#"><span class="glods"></span><span class="get-money">领淘金币抵钱</span></a>
<a class="vip-home" href="#">会员俱乐部</a>
</div>
</div>
<!--login-->
<div class="login">
<a class="login-btn" href="#"><i class="fa fa-user fa-user-loc"></i>登陆</a>
<a class="login-btn free" href="#">免费注册</a>
<a class="login-btn free" href="#">免费开店</a>
</div>
</div>
<!--service-->
<div class="service">
<h3>便民服务</h3>
<div id="service-1" class="service-cell service-z">
<h5 class="service-i"><img src="/myweb/xinyi/Public/img/phone.png" /></h5>
<h6>话费</h6>
<i class="fa fa-angle-down"></i>
</div>
<div id="service-2" class="service-cell service-z">
<h5 class="service-i"><img src="/myweb/xinyi/Public/img/game.png" /></h5>
<h6>游戏</h6>
<i class="fa fa-angle-down"></i>
</div>
<div id="service-3" class="service-cell service-z">
<h5 class="service-i"><img src="/myweb/xinyi/Public/img/plane.png" /></h5>
<h6>旅行</h6>
<i class="fa fa-angle-down"></i>
</div>
<div id="service-4" class="service-cell service-z">
<h5 class="service-i"><img src="/myweb/xinyi/Public/img/save.png" /></h5>
<h6>保险</h6>
<i class="fa fa-angle-down"></i>
</div>
<div class="service-cell">
<h5 class="service-i"><img src="/myweb/xinyi/Public/img/18.png" /></h5>
<h6>彩票</h6>
</div>
<div class="service-cell">
<h5 class="service-i"><img src="/myweb/xinyi/Public/img/move.png" /></h5>
<h6>电影</h6>
</div>
<div class="service-cell">
<h5 class="service-i"><img src="/myweb/xinyi/Public/img/eat.png" /></h5>
<h6>点外卖</h6>
</div>
<div class="service-cell">
<h5 class="service-i"><img src="/myweb/xinyi/Public/img/money.png" /></h5>
<h6>理财</h6>
</div>
<div class="service-cell">
<h5 class="service-i"><img src="/myweb/xinyi/Public/img/book.png" /></h5>
<h6>电子书</h6>
</div>
<div class="service-cell">
<h5 class="service-i"><img src="/myweb/xinyi/Public/img/miusc.png" /></h5>
<h6>音乐</h6>
</div>
<div class="service-cell">
<h5 class="service-i"><img src="/myweb/xinyi/Public/img/bag.png" /></h5>
<h6>水电煤</h6>
</div>
<div class="service-cell">
<h5 class="service-i"><img src="/myweb/xinyi/Public/img/....png" /></h5>
<h6>请期待</h6>
</div>
</div>
<!--service-box-->
<div id="service-box-1" class="service-box">
<div class="service-head">
<a href="#">话费充值</a>
<a href="#">流量充值</a>
<span class="fa fa-times"></span>
</div>
<div class="feihua-in">
<span>号码</span>
<input class="tell-num" type="text" placeholder="手机号、固话号"/>
</div>
<div class="feihua-in">
<span>面值</span>
<input class="money-in" type="text" value="50" />
<span class="fa fa-angle-down sel-money"></span>
</div>
<div class="gary-text"><span>售价 ¥ </span><span class="orange">49-49.8</span></div>
<div style="margin-top: 10px">
<a href="#" class="now-chongzhi">立即充值</a>
<a href="#" class="now-chongzhi dingqi">定期充值</a>
<a href="#">3G急速上网卡</a>
</div>
</div>
<div id="service-box-2" class="service-box">
<div class="service-head">
<a href="#">话费充值</a>
<a href="#">流量充值</a>
<span class="fa fa-times"></span>
</div>
<div class="feihua-in">
<span>号码</span>
<input class="tell-num" type="text" placeholder="手机号、固话号"/>
</div>
<div class="feihua-in">
<span>面值</span>
<input class="money-in" type="text" value="50" />
<span class="fa fa-angle-down sel-money"></span>
</div>
<div class="gary-text"><span>售价 ¥ </span><span class="orange">49-49.8</span></div>
<div style="margin-top: 10px">
<a href="#" class="now-chongzhi">立即充值</a>
<a href="#" class="now-chongzhi dingqi">定期充值</a>
<a href="#">3G急速上网卡</a>
</div>
</div>
<div id="service-box-3" class="service-box">
<div class="service-head">
<a href="#">话费充值</a>
<a href="#">流量充值</a>
<span class="fa fa-times"></span>
</div>
<div class="feihua-in">
<span>号码</span>
<input class="tell-num" type="text" placeholder="手机号、固话号"/>
</div>
<div class="feihua-in">
<span>面值</span>
<input class="money-in" type="text" value="50" />
<span class="fa fa-angle-down sel-money"></span>
</div>
<div class="gary-text"><span>售价 ¥ </span><span class="orange">49-49.8</span></div>
<div style="margin-top: 10px">
<a href="#" class="now-chongzhi">立即充值</a>
<a href="#" class="now-chongzhi dingqi">定期充值</a>
<a href="#">3G急速上网卡</a>
</div>
</div>
<div id="service-box-4" class="service-box">
<div class="service-head">
<a href="#">话费充值</a>
<a href="#">流量充值</a>
<span class="fa fa-times"></span>
</div>
<div class="feihua-in">
<span>号码</span>
<input class="tell-num" type="text" placeholder="手机号、固话号"/>
</div>
<div class="feihua-in">
<span>面值</span>
<input class="money-in" type="text" value="50" />
<span class="fa fa-angle-down sel-money"></span>
</div>
<div class="gary-text"><span>售价 ¥ </span><span class="orange">49-49.8</span></div>
<div style="margin-top: 10px">
<a href="#" class="now-chongzhi">立即充值</a>
<a href="#" class="now-chongzhi dingqi">定期充值</a>
<a href="#">3G急速上网卡</a>
</div>
</div>
</div>
</div>
</div>
<!--main-->
<div class="sub-nav">
<input type="button" class="more-btn" value="更多"/>
<!--sub-nav-cell-->
<div class="sub-nav-cell">
<h3 class="orange">主题市场</h3>
<ul class="sub-nav-box">
<li><a href="#">爱美女人</a></li>
<li><a href="#">品质男人</a></li>
<li><a href="#">淘宝二手</a></li>
<li><a href="#">全球购</a></li>
<li><a href="#">品牌街</a></li>
<li><a href="#">汽车生活</a></li>
<li><a href="#">花嫁新娘</a></li>
<li><a href="#">孕婴童</a></li>
<li><a href="#">同城便民</a></li>
<li><a href="#">爱么装</a></li>
<li><a href="#">运动户外</a></li>
<li><a href="#">家用电器</a></li>
<li><a href="#">狠爱</a></li>
<li><a href="#">网络游戏</a></li>
<li><a href="#">潮流设计</a></li>
<li><a href="#">淘宝星愿</a></li>
</ul>
</div>
<div class="sub-nav-cell">
<h3 class="green-sp">特色购物</h3>
<ul class="sub-nav-box sp">
<li><a href="#">爱美女人</a></li>
<li><a href="#">品质男人</a></li>
<li><a href="#">淘宝二手</a></li>
<li><a href="#">全球购</a></li>
<li><a href="#">品牌街</a></li>
<li><a href="#">汽车生活</a></li>
<li><a href="#">花嫁新娘</a></li>
<li><a href="#">孕婴童</a></li>
<li><a href="#">同城便民</a></li>
<li><a href="#">爱么装</a></li>
<li><a href="#">运动户外</a></li>
<li><a href="#">家用电器</a></li>
<li><a href="#">狠爱</a></li>
<li><a href="#">网络游戏</a></li>
<li><a href="#">潮流设计</a></li>
<li><a href="#">淘宝星愿</a></li>
</ul>
</div>
<div class="sub-nav-cell">
<h3 class="red-now">当前热点</h3>
<ul class="sub-nav-box now">
<li><a href="#">爱美女人</a></li>
<li><a href="#">品质男人</a></li>
<li><a href="#">淘宝二手</a></li>
<li><a href="#">全球购</a></li>
<li><a href="#">品牌街</a></li>
<li><a href="#">汽车生活</a></li>
<li><a href="#">花嫁新娘</a></li>
<li><a href="#">孕婴童</a></li>
<li><a href="#">同城便民</a></li>
<li><a href="#">爱么装</a></li>
<li><a href="#">运动户外</a></li>
<li><a href="#">家用电器</a></li>
<li><a href="#">狠爱</a></li>
<li><a href="#">网络游戏</a></li>
<li><a href="#">潮流设计</a></li>
<li><a href="#">淘宝星愿</a></li>
</ul>
</div>
<div class="sub-nav-cell">
<h3 class="blue-more">更多精彩</h3>
<ul class="sub-nav-box more">
<li><a href="#">爱美女人</a></li>
<li><a href="#">品质男人</a></li>
<li><a href="#">淘宝二手</a></li>
<li><a href="#">全球购</a></li>
<li><a href="#">品牌街</a></li>
<li><a href="#">汽车生活</a></li>
<li><a href="#">花嫁新娘</a></li>
<li><a href="#">孕婴童</a></li>
<li><a href="#">同城便民</a></li>
<li><a href="#">爱么装</a></li>
<li><a href="#">运动户外</a></li>
<li><a href="#">家用电器</a></li>
<li><a href="#">狠爱</a></li>
<li><a href="#">网络游戏</a></li>
<li><a href="#">潮流设计</a></li>
<li><a href="#">淘宝星愿</a></li>
</ul>
</div>
</div>
<!--main-->
<div class="main">
<div class="main-left">
<div class="main-title">
<h1>精彩推荐</h1>
</div>
<?php if(is_array($products)): foreach($products as $key=>$v): ?><div class="product-box">
<div class="inner-info">
<div>
<span class="line-f40">|</span>
<span class="text-title"><?php echo ($v["shopname"]); ?></span>
<span class="share-weitao">
<span class="line-f40">+</span>
<a href="#">更多信息</a>
</span>
</div>
<div class="inner-left">
<img style="max-width:168px; max-height:130px" src="/myweb/xinyi<?php echo ($v["imgurl"]); ?>" />
<h1 style="color:#f00">¥<?php echo ($v["price"]); ?></h1>
<span><?php echo ($v["name"]); ?></span>
</div>
<div class="inner-right">
<div>
<a href="#">
<img src="/myweb/xinyi/Public/img/shooes.jpg" />
<p>春夏美鞋</p>
</a>
<a href="#">
<img src="/myweb/xinyi/Public/img/c.gif" />
<p>春季亮色搭</p>
</a>
</div>
<table class="tab-inner">
<tr><td><a href="#">降价通知</a></td>
<td><a href="#">立即购买</a></td>
</tr>
<tr><td><a href="#">加入收藏</a></td>
<td><a href="#">加入购物车</a></td>
</tr>
</table>
</div>
</div>
</div><?php endforeach; endif; ?>
</div>
<div class="main-right">
<div class="time-go">
<div class="time-cell">
<h1><a href="#" class="text_shadow">我收藏的店铺上新</a><span class="now-news">更新<span class="">3</span></span></h1>
<div class="inner-show">
<a href="#">
<img src="/myweb/xinyi/Public/img/inner1.png">
<p class="price-info">¥118.00</p>
</a>
<a href="#">
<img src="/myweb/xinyi/Public/img/inner2.png">
<p>¥118.00</p>
</a>
<a href="#">
<img src="/myweb/xinyi/Public/img/inner3.png">
<p>¥118.00</p>
</a>
<h3><a href="$">更多店铺上新</a><i class="fa fa-angle-right"></i></h3>
</div>
</div>
</div>
<!--fix-right-->
</div>
</div>
<!--main-bottom-->
<div class="main-bottom">
<div class="sub-bottom-nav">
<h1>热卖单品</h1>
<div class="sub-nav-info">
<a href="#">链接1</a>|
<a href="#">链接2</a>|
<a href="#">链接3</a>|
<a href="#">链接4</a>|
<a href="#">链接5</a>|
<a href="#">链接6</a>|
<a href="#">链接7</a>|
<a href="#">链接8</a>
</div>
</div>
<div class="single-sale">
<a href="#">
<img src="/myweb/xinyi/Public/img/sing1.jpg" />
<h3>褶皱立领雪纺衫</h3>
</a>
<p><span class="orange">¥ </span><span class="orange">129.00</span><span class="send-free">包邮</span></p>
<p class="gary-text"><span>¥ </span><span><del>399.00</del></span> | <span>月消29件</span></p>
</div>
</div>
<!--help-->
<div class="help">
<div class="help-info">
<h1><img src="/myweb/xinyi/Public/img/help4.png"><span class="help-text">消费者保障</span></h1>
<a class="help-a" href="#">保障范围</a>
<a class="help-a" href="#">退货退款流程</a>
<a class="help-a" href="#">服务中心</a>
<a class="help-a" href="#">更多特色服务</a>
</div>
<div class="help-info">
<h1><img src="/myweb/xinyi/Public/img/help1.png"><span class="help-text">新手上路</span></h1>
<a class="help-a" href="#">新手专区</a>
<a class="help-a"v href="#">消费警示</a>
<a class="help-a" href="#">交易安全</a>
<a class="help-a margin-r" href="#">24小时在线帮助</a>
</div>
<div class="help-info">
<h1><img src="/myweb/xinyi/Public/img/help2.png"><span class="help-text">付款方式</span></h1>
<a class="help-a-litter" href="#">支付宝快捷支付</a>
<a class="help-a-litter" href="#">支付宝卡(现金)付款</a>
<a class="help-a-litter" href="#">支付宝余额付款</a>
<a class="help-a" href="#">货到付款</a>
</div>
<div class="help-info">
<h1><img src="/myweb/xinyi/Public/img/help3.png"><span class="help-text">淘宝特色</span></h1>
<a class="help-a" href="#">淘宝指数</a>
<a class="help-a" href="#">淘公仔</a>
<a class="help-a" href="#">手机淘宝</a>
<a class="help-a" href="#">旺信</a>
</div>
</div>
<!--footer-->
<div class="footer">
<div class="footer-right">
<div class="footer-nav">
<a href="#">阿里巴巴集团</a>|
<a href="#">阿里巴巴国际站</a>|
<a href="#">阿里巴巴中国站</a>|
<a href="#">全球速卖通</a>|
<a href="#">淘宝网</a>|
<a href="#">天猫</a>|
<a href="#">聚划算</a>|
<a href="#">一淘</a>|
<a href="#">阿里妈妈</a>|
<a href="#">阿里云计算</a>|
<a href="#">云OS</a>|
<a href="#">万网</a>|
<a href="#">支付宝</a>|
<a href="#">来往</a>
</div>
<div class="about-tao">
<a href="#">关于淘宝</a>
<a href="#">合作伙伴</a>
<a href="#">营销中心</a>
<a href="#">廉正举报</a>
<a href="#">联系客服</a>
<a href="#">开放平台</a>
<a href="#">诚征英才</a>
<a href="#">联系我们</a>
<a href="#">网站地图</a>
<a href="#">法律声明</a>
<span class="gary-text">© 2014 Taobao.com 版权所有</span>
</div>
<a class="app-in-grd" href='itms-services://?action=download-manifest&url=https://i.s.manxapp.com/app/manbetx.plist'>下载IOS</a>
<p class="gary-text"><span>网络文化经营许可证:文网文[2010]040号</span>|<span>增值电信业务经营许可证:浙B2-20080224-1</span>|<span>信息网络传播视听节目许可证:1109364号</span></p>
<div class="some-info">
<img src="/myweb/xinyi/Public/img/some.png" />
</div>
</div>
<div class="tao-man">
<img src="/myweb/xinyi/Public/img/tao-man.png" />
</div>
</div>
<!--backtoTop1-->
<!--兼容所有现代浏览器同时包括 ie6/7/8/9 (ie6会有一点点抖动)-->
<div class="backtoTop" id="backToTop1">
<div id="backToTop-up" class="up-back"><i class="fa fa-angle-up"></i></div>
<div id="backToTop-down" class="down-back"><i class="fa fa-angle-down"></i></div>
</div>
<script src="/myweb/xinyi/Public/js/jquery_1.9.js"></script>
<script src="/myweb/xinyi/Public/js/main.js"></script>
<script src="/myweb/xinyi/Public/js/img-show.js"></script>
</body>
</html><file_sep>/Apps/Common/Common/function.php
<?php
function compute_level($count,$step) {
$level = 0;
$num = $count;
while ( $num > 0 ) {
$level ++;
$num = $num - round ( pow ( $step, $level ) + 0.5 );
}
return $level;
}
function result_user($user) {
unset ( $user ['regtime'] );
unset ( $user ['loginword'] );
return $user;
}
/**
* logininfo表插入数据
* @param unknown $id
*/
function insertLogin($id) {
if ($id && $id != "") {
$loginDao = M ( 'ulogininfo' );
$logininfo = array (
'user_id' => $id,
'clientIP' => get_client_ip (),
'logintime' => time ()
);
$loginDao->add ( $logininfo );
}
}
/**
* userinfo表更新数据
* @param unknown $userDao
* @param unknown $post_user
* @param unknown $sql_user
*/
function upUser($userDao,$post_user,&$sql_user){
$sql_user ['level'] = compute_level ( ++ $sql_user ['logincount'], 1.3 );
$sql_user ['lastlogintime'] = $sql_user ['logintime'];
$sql_user ['logintime'] = time ();
return $userDao->save( $sql_user );
}
/**
* userinfo表插入数据
* @param unknown $post_user
* @param unknown $userDao
*/
function insertUser(&$post_user,$userDao){
$post_user ['level'] = 1;
$post_user ['logincount'] = 1;
$post_user ['regtime'] = time ();
$post_user ['logintime'] = $post_user ['regtime'];
$post_user ['lastlogintime'] = $post_user ['regtime'];
return $userDao->add ( $post_user );
}
/**
* post提交数据
* @param unknown $url
* @param unknown $data
* @return mixed
*/
function vpost($url,$data){ // 模拟提交数据函数
$curl = curl_init(); // 启动一个CURL会话
curl_setopt($curl, CURLOPT_URL, $url); // 要访问的地址
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, 0); // 对认证证书来源的检查
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, 1); // 从证书中检查SSL加密算法是否存在
curl_setopt($curl, CURLOPT_USERAGENT, $_SERVER['HTTP_USER_AGENT']); // 模拟用户使用的浏览器
curl_setopt($curl, CURLOPT_FOLLOWLOCATION, 1); // 使用自动跳转
curl_setopt($curl, CURLOPT_AUTOREFERER, 1); // 自动设置Referer
curl_setopt($curl, CURLOPT_POST, 1); // 发送一个常规的Post请求
curl_setopt($curl, CURLOPT_POSTFIELDS, $data); // Post提交的数据包
curl_setopt($curl, CURLOPT_TIMEOUT, 30); // 设置超时限制防止死循环
curl_setopt($curl, CURLOPT_HEADER, 0); // 显示返回的Header区域内容
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1); // 获取的信息以文件流的形式返回
//https请求 不验证证书和host
// curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false);
// curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false);
$result = curl_exec($curl); // 执行操作
if (curl_errno($curl)) {
echo 'Errno'.curl_error($curl);//捕抓异常
}
curl_close($curl); // 关闭CURL会话
return $result; // 返回数据
}
function vget($url, $params) {
if($params){
$url = ($url . "?" . $params);
}
$ch = curl_init ();
curl_setopt ( $ch, CURLOPT_URL, $url );
curl_setopt ( $ch, CURLOPT_SSL_VERIFYPEER, false );
curl_setopt ( $ch, CURLOPT_SSL_VERIFYHOST, false );
$result = curl_exec ( $ch );
return $result;
}<file_sep>/Apps/Admin/Controller/WelcomeController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
class WelcomeController extends AuthController {
public function index() {
$images=M("welcome")->select();
$this->images=$images;
$this->imgnum=sizeof($images);
$this->display ();
}
public function add() {
$upload = new \Think\Upload (); // 实例化上传类
$upload->maxSize = 3145728; // 设置附件上传大小
$upload->exts = array ( 'jpg','png' ); // 设置附件上传类型
$upload->rootPath = './UpLoads/';
$upload->savePath = '/welcome/images/'; // 设置附件上传目录
// 上传单个文件
$info = $upload->uploadOne($_FILES['proimg1']);
if(!$info) {// 上传错误提示错误信息
$this->error($upload->getError());
}else{// 上传成功 获取上传文件信息
$imageurl = '/UpLoads'.$info['savepath'].$info['savename'];
if(!M("welcome")->add(array('imageurl'=>$imageurl))){
$this->error("添加图像文件失败!");
}else{
import("Fly.MyImage",".");
\MyImage::thumb(".".$imageurl, 480, 800);
$this->redirect("/Admin/Welcome");
}
}
}
public function del(){
if(!IS_POST) $this->error("页面不存在!");
$id=I('post.id','','htmlspecialchars');
if(M("welcome")->where(array('id'=>$id))->delete()){
$this->redirect("/Admin/Welcome");
}else{
$this->error("删除图像失败!");
}
}
}<file_sep>/Apps/API/Controller/ProductController.class.php
<?php
namespace API\Controller;
use Think\Controller\RestController;
class ProductController extends RestController {
protected $allowMethod = array('get','post','put','delete'); // REST允许的请求类型列表
protected $allowType = array('json'); // REST允许请求的资源类型列表
public function index() {
header ( 'Cache-Control:max-age=0' );
header ( 'Content-Type:text/html;charset=utf-8 ', true );
header ( 'Last-Modified: ' . gmdate ( 'D, d M Y H:i:s', time () ) . ' GMT', true );
$data = D ( 'ProductAll' )->relation(true)->select();
$this->response($data,'json');
}
public function type(){
header ( 'Cache-Control:max-age=0' );
header ( 'Content-Type:text/html;charset=utf-8 ', true );
header ( 'Last-Modified: ' . gmdate ( 'D, d M Y H:i:s', time () ) . ' GMT', true );
$data = D ( 'ProductAll' )->where(array('ptype_id'=>I('type')))->relation(true)->select();
$this->response($data,'json');
}
public function id(){
header ( 'Cache-Control:max-age=0' );
header ( 'Content-Type:text/html;charset=utf-8 ', true );
header ( 'Last-Modified: ' . gmdate ( 'D, d M Y H:i:s', time () ) . ' GMT', true );
$data = D ( 'ProductAll' )->where(array('id'=>I('id')))->relation(true)->select();
$this->response($data,'json');
}
}<file_sep>/Apps/Admin/Controller/AttrController.class.php
<?php
namespace Admin\Controller;
use Think\Controller;
class AttrController extends AuthController {
/**
* 商品属性页面
*/
public function index(){
$this->pattr=M('pattr')->select();
$this->display();
}
/**
* 添加商品属性
*/
public function add(){
if(!IS_POST) $this->error("页面不存在!");
if($_POST['name']==null||$_POST['color']==null){
$this->error("传入参数错误!");
}
$pattrDao = M('pattr');
if($pattrDao->add($_POST)){
$this->redirect("/Admin/Attr");
}else{
$this->error($pattrDao->getError());
}
}
/**
* 删除商品属性
*/
public function del(){
if (! IS_POST) $this->error("页面不存在!");
$pattrDao = M('pattr');
if($pattrDao->where(array($_POST))->delete()){
$this->redirect("/Admin/Attr");
}else{
$this->error($pattrDao->getError());
}
}
}<file_sep>/Apps/Cls/User.class.php
<?php
class User{
/**
* 验证数据库中的帐号是否存在。
* 返回值:帐号不存在近回NULL否则返回帐号。
* @param string $tabla 数据表名
* @param string $userName 帐号
* @param string $name_id 帐号在数据表中的字段名
*/
public static function verifyName($tabla='user',$userName,$name_filed="loginname"){
return M($tabla)->field($name_filed)->where(array($name_filed=>$userName))->find();
}
/**
* 验证数据库中的帐号和密码是否正确。
* 返回值:-1,验证帐号不存在;0,验证密码不正确;验证通过返回用户在数据表中的记录。
* @param string $tabla 数据表名
* @param string $userName 帐号
* @param string $userPswd 密码
* @param string $name_id 帐号在数据表中的字段名
* @param string $pwsd_id 密码在数据表中的字段名
*
*/
public static function verifyUser($tabla='user',$userName,$userPswd,$name_filed="loginname",$pwsd_filed="loginword"){
$data=M($tabla)->where(array($name_filed=>$userName))->find();
if(!$data) return -1;
if($data[$pwsd_filed]!=md5($userPswd)) return 0;
return $data;
}
/**
* 向数据表$table中插入一条数据$data。
* 成功返回插入的数据,失败返回false。
* @param unknown $table
* @param unknown $data
*/
public static function insertUser($table='user',$data){
//初始化其它要插入的数据
$data['level'] = 1;
$data['logincount'] = 1;
$data['regtime'] = time();
//暂时使用用户名作为昵称。
$data['nickname'] = $data ['nickname'] != null ? $data ['nickname'] : $data ['loginname'];
//密码进行md5加密。
$data['loginword'] = md5($data['loginword']);
$ret=M($table)->add($data);
if($ret){
$data['id']=$ret;
return $data;
}else{
return false;
}
}
/**
* 插入帐号登陆信息。成功返回插入信息,失败返回false。
* @param unknown $table
* @param unknown $id
*/
public static function insertLog($table='user_log',$id,$user_id='user_id'){
$data = array (
$user_id => $id,
'logintime' => time (),
'loginIP'=>get_client_ip(),
);
if(M($table)->add($data)){
return $data;
}else{
return false;
}
}
/**
* 保存cookie
* @param unknown $data
* @param unknown $log
* @param number $time
*/
public static function saveCookie($data,$log,$time=3600){
cookie ( 'loginname', $data ['loginname'], $time );
cookie ( 'nickname', $data ['nickname'] != null ? $data ['nickname'] : $data ['loginname'], $time );
cookie ( 'level', $data ['level'], $time );
cookie ( 'logincount', $data ['logincount'] );
cookie ( 'id', $log ['user_id'], $time );
cookie ( 'logintime', $log ['logintime'], $time );
cookie ( 'loginIP', $log ['loginIP'], $time );
}
/**
* 保存session
* @param unknown $data
* @param unknown $log
*/
public static function saveSession($data,$log){
session ( 'loginname', $data ['loginname'] );
session ( 'nickname', $data ['nickname'] != null ? $data ['nickname'] : $data ['loginname'] );
session ( 'level', $data ['level'] );
session ( 'logincount', $data ['logincount'] );
session ( 'id', $log ['user_id'] );
session ( 'logintime', $log ['logintime'] );
session ( 'loginIP', $log ['loginIP'] );
}
/**
* App返回包含用户信息的json字符串
* @param unknown $data
* @param unknown $log
*/
public static function echoUserJson($data,$log){
$data ['nickname']= $data ['nickname'] != null ? $data ['nickname'] : $data ['loginname'];
$data['user_id'] = $log ['user_id'] ;
$data ['logintime']= $log ['logintime'];
unset($data['loginword']);
unset($data['openid']);
unset($data['regtime']);
unset($data['lock']);
echo json_encode($data);
}
/**
* 计算用户等级
* @param unknown $count
* @param unknown $step
* @return number
*/
public static function computeLevel($count,$step=1.3) {
$level = 0;
$num = $count;
while ( $num > 0 ) {
$level ++;
$num = $num - round ( pow ( $step, $level ) + 0.5 );
}
return $level;
}
/**
* 更新用户等级
* @param unknown $userDao
* @param unknown $post_user
* @param unknown $sql_user
*/
public static function upUserLevel($table='user',&$data){
$data['level']= \User::computeLevel( ++ $data ['logincount']);
return M($table)->save($data);
}
}<file_sep>/Runtime/Cache/Admin/ec0657704c9aaed9286a02f12c56ca11.php
<?php if (!defined('THINK_PATH')) exit();?><!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>宇翔科技后台管理-移动端管理</title>
<link rel="stylesheet" type="text/css" href="/myweb/xinyi/Apps/Admin/Public/css/welcome.css" />
<link rel="stylesheet" type="text/css" href="/myweb/xinyi/Apps/Admin/Public/css/font-awesome.min.css" />
<script type="text/javascript"> var imgnum = <?php echo ($imgnum); ?>;</script>
<style type="text/css">
.imgBox {
position: absolute;
clear: both;
list-style-type: none;
width: <?php echo ($imgnum*480); ?>px;
}
.currentNum {
position: absolute;
left: 50%;
top: 780px;
width: 480px;
height: 11px;
margin-left: -<?php echo ($imgnum*7); ?>px;
}
</style>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/jquery-1.9.0.min.js"></script>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/admin-welcome.js"></script>
</head>
<body>
<div class="crumb-wrap">
<span class="icon-font">  <a href="<?php echo U('/Admin');?>">首页</a></span> <span class="crumb-step">> <a href="#">移动端首页广告管理</a></span>
</div>
<div class="main-wrap">
<div class="result-wrap">
<div class="showimgs">
<div class="content">
<ul class="imgBox">
<?php if(is_array($images)): foreach($images as $key=>$v): ?><li>
<a href="javascript:delDlg('<?php echo ($v["id"]); ?>')"><img src="<?php echo U($v['imageurl']);?>"></a>
</li><?php endforeach; endif; ?>
</ul>
<div class="currentNum">
<span class="imgNum mark-color"></span>
<?php for($i=1;$i<$imgnum;$i++){?>
<span class="imgNum"></span>
<?php } ?>
</div>
<div class="control to-left">
<i class="fa fa-angle-left"></i>
</div>
<!-- div class="control to-center"><a>删除</a></div-->
<div class="control to-right">
<i class="fa fa-angle-right"></i>
</div>
<div class="dlg-content1">
<form name="delFrom" id="delFrom" accept-charset="utf-8" action="<?php echo U('/Admin/welcome/del');?>" method="post">
<input type="hidden" value="" name="id" id="imageID">
你确定要删除该图像吗
<table width="100%">
<tr>
<td><input type="submit" class="btn btn-primary" value="删除"></td>
<td><input type="button" class="btn btn-primary" onclick="javascript:closeDlg()" value="取消"></td>
</tr>
</table>
</form>
</div>
</div>
</div>
<form action="<?php echo U('/Admin/Welcome/add');?>" method="post" id="addFrom" name="addFrom" enctype="multipart/form-data">
<table class="insert-tab" width="100%">
<tbody>
<tr>
<th><span class="require-red">*</span>上传图像:</th>
<td><input class="common-text" name="proimg1" id="proimg1" type="file" accept=".jpg,.png"><span class="require-red">支持格式PNG、JPG,分辨率:480*800。</span> <!--<input class="btn btn6" type="submit" onclick="submitForm('/jscss/admin/design/upload')" value="上传图片"/>-->
</td>
<td><input type="submit" class="btn btn-primary btn6 mr10" value="上传" id="add"></td>
</tr>
</tbody>
</table>
</form>
</div>
</div>
<!-- 添加菜单 Start-->
<script src="/myweb/xinyi/Apps/Admin/Public/js/img-show.js"></script>
</body>
</html><file_sep>/Apps/Common/Model/HomeShopModel.class.php
<?php
namespace Common\Model;
use Think\Model\RelationModel;
class HomeShopModel extends RelationModel {
// 定义主表
protected $tableName = 'shop_home';
// 定义关联关系
protected $_link = array (
'shop' => array (
'mapping_type' => self::HAS_ONE,
'class_name' => 'shop', // 要关联的模型类名
'foreign_key' => 'id', // 关联的外键名称
'as_fields' => 'shopname'
),
'imgurl' => array(
'mapping_type' => self::HAS_ONE,
'class_name' => 'shop_imgurl', //要关联的模型类名
'foreign_key' => 'shop_id', //关联的外键名称
// 'mapping_name' => 'articles',
'mapping_name' => 'imgurl',
// 'mapping_fields'=> 'imageurl',
'as_fields'=>'imgurl',
),
);
}<file_sep>/Apps/Admin/Public/js/admin-welcome.js
$(document).ready(function() {
$('#add').click(function() {
if ($('#proimg1').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#proimg1').focus();
return false;
}
$('#addFrom').submit();
});
});
function delDlg(s1) {
$('.dlg-content1').fadeIn(100);
// $('.dlg-mask').fadeIn(100);
// 设置初始值
document.getElementById("imageID").value = s1;
}
function closeDlg() {
$('.dlg-content1').fadeOut(100);
}
<file_sep>/Runtime/Cache/Admin/707b16be43de1247e1cd48ae9a29332d.php
<?php if (!defined('THINK_PATH')) exit();?>
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>宇翔科技后台管理</title>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/jquery-1.9.0.min.js"></script>
<script type="text/javascript"> var ajaxurl= '<?php echo U("/Admin/Type/del","","");?>'</script>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/admin-type.js"></script>
</head>
<body>
<div class="crumb-wrap">
<span class="icon-font">  <a href="<?php echo U('/Admin');?>">首页</a></span>
<span class="crumb-step">> <a href="#">商品分类</a></span>
</div>
<div class="main-wrap">
<div class="result-wrap">
<table class="admin-tab" width="900px">
<tbody align="right">
<tr style="background:#e8e8e8">
<th width=%25>分类名称</th>
<th width=%25>分类层次</th>
<th width=%25>排列顺序</th>
<th width=%25>执行操作</th>
</tr>
<?php if(is_array($ptype)): foreach($ptype as $key=>$v): ?><tr>
<th><?php echo ($v["ptype_name"]); ?></th>
<th><?php echo ($v["pid"]); ?></th>
<th><?php echo ($v["order"]); ?></th>
<th><a href="javascript:postdel('<?php echo ($v["id"]); ?>','<?php echo ($v["name"]); ?>','<?php echo ($v["pid"]); ?>','<?php echo ($v["order"]); ?>');"><i class="icon-font">删除</i></a></th>
</tr><?php endforeach; endif; ?>
</tbody>
</table>
<br>
<form name="addFrom" id="addFrom" accept-charset="utf-8" action="<?php echo U('/Admin/Type/add');?>" method="post">
<table class="admin-tab" width="900px">
<tbody>
<tr>
<th style="width:838px;background:#e8e8e8" colspan="2">添加分类</th>
</tr>
<tr>
<th width = "200px"><span class="require-red">*</span>分类名称:</th>
<td>
<input class="common-text" id="ptype_name" name="ptype_name" size="40" value="" type="text">
</td>
</tr>
<tr>
<th><span class="require-red">*</span>分类层次:</th>
<td>
<input class="common-text" id="pid" name="pid" size="40" value="0" type="text">
</td>
</tr>
<tr>
<th><span class="require-red">*</span>排列顺序:</th>
<td>
<input class="common-text" id="order" name="order" size="40" value="100" type="text">
</td>
</tr>
<tr>
<th></th>
<td>
<input type="submit"class="btn btn-primary btn6 mr10" value="添加" id="add">
<input type="reset" class="btn btn-primary btn6 mr10" value="重置">
</td>
</tr>
</tbody>
</table>
</form>
</div>
</div>
</body>
</html><file_sep>/Runtime/Cache/Admin/1c30f6ab002a9149cc7388ff45b634b3.php
<?php if (!defined('THINK_PATH')) exit();?>
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>宇翔科技后台管理</title>
<link rel="stylesheet" type="text/css" href="/myweb/xinyi/Apps/Admin/Public/css/node.css" />
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/jquery-1.9.0.min.js"></script>
<script type="text/javascript"> var delurl= '<?php echo U("/Admin/Node/del");?>'</script>
<script type="text/javascript"> var statusurl= '<?php echo U("/Admin/Node/status");?>'</script>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/admin-node.js"></script>
</head>
<body>
<div class="crumb-wrap">
<span class="icon-font">  <a href="<?php echo U('/Admin');?>">首页</a></span>
<span class="crumb-step">> <a href="#">节点管理</a></span>
</div>
<div class="main-wrap">
<div class="result-wrap">
<div class="apps-title">网站目录<a href="javascript:addDlg('','0','1');">+</a>
<?php if(is_array($node)): foreach($node as $key=>$v): ?><div class="apps">
<div class="app"><?php echo ($v["remark"]); ?><!--
--><a href="javascript:addDlg('<?php echo ($v["remark"]); ?>','<?php echo ($v["id"]); ?>','2');">+</a><!--
--><?php if($v['child']==null): ?><a href="javascript:delDlg('<?php echo ($v["id"]); ?>','<?php echo ($v["name"]); ?>','<?php echo ($v["remark"]); ?>','<?php echo ($v["status"]); ?>','<?php echo ($v["pid"]); ?>','<?php echo ($v["level"]); ?>');">-</a><!--
--><?php endif; ?><a href="javascript:editDlg('<?php echo ($v["id"]); ?>','<?php echo ($v["name"]); ?>','<?php echo ($v["remark"]); ?>','1','<?php echo ($v["pid"]); ?>','<?php echo ($v["level"]); ?>','');">E</a>
</div>
<?php if(is_array($v["child"])): foreach($v["child"] as $key=>$c): ?><div class="controls">
<div class="control"><?php echo ($c["remark"]); ?><!--
--><a href="javascript:addDlg('<?php echo ($c["remark"]); ?>','<?php echo ($c["id"]); ?>','3');">+</a><!--
--><?php if($c['child']==null): ?><a href="javascript:delDlg('<?php echo ($c["id"]); ?>','<?php echo ($c["name"]); ?>','<?php echo ($c["remark"]); ?>','<?php echo ($v["status"]); ?>','<?php echo ($c["pid"]); ?>','<?php echo ($c["level"]); ?>');">-</a><!--
--><?php endif; ?><a href="javascript:editDlg('<?php echo ($c["id"]); ?>','<?php echo ($c["name"]); ?>','<?php echo ($c["remark"]); ?>','1','<?php echo ($c["pid"]); ?>','<?php echo ($c["level"]); ?>','<?php echo ($v["remark"]); ?>');">E</a>
</div>
<?php if(is_array($c["child"])): foreach($c["child"] as $key=>$m): ?><div class="method"><?php echo ($m["remark"]); ?><!--
--><a href="javascript:delDlg('<?php echo ($m["id"]); ?>','<?php echo ($m["name"]); ?>','<?php echo ($m["remark"]); ?>','<?php echo ($v["status"]); ?>','<?php echo ($m["pid"]); ?>','<?php echo ($m["level"]); ?>');">-</a><!--
--><a href="javascript:editDlg('<?php echo ($m["id"]); ?>','<?php echo ($m["name"]); ?>','<?php echo ($m["remark"]); ?>','1','<?php echo ($m["pid"]); ?>','<?php echo ($m["level"]); ?>','<?php echo ($c["remark"]); ?>');">E</a>
</div><?php endforeach; endif; ?>
</div><?php endforeach; endif; ?>
</div><?php endforeach; endif; ?>
</div>
</div>
</div>
<!-- 弹出菜单 Start-->
<div class="node-mask"></div>
<!-- 添加菜单 Start-->
<div class="node-dlg-add">
<form name="addFrom" id="addFrom" accept-charset="utf-8" action="<?php echo U('Admin/Node/add');?>" method="post">
<table class="admin-tab" width="450px">
<tbody>
<div class="node-dlg-title">添加节点
<div class="node-dlg-title-close"><a href="javascript:closeAddDlg();" title="关闭" >×</a></div>
</div>
<tr>
<th><span class="require-red">*</span>节点名称:</th>
<td>
<input class="common-text" type="text" id="node_name" name="node_name" size="20" value="">
</td>
</tr>
<tr>
<th><span class="require-red">*</span>节点描述:</th>
<td>
<input class="common-text" type="text" id="node_remark" name="node_remark" size="40" value="">
</td>
</tr>
<tr>
<th><span class="require-red">*</span>是否启用:</th>
<td>
<input class="common-text" id="node_status" name="node_status" value="1" type="radio" checked = "checked">开启
<input class="common-text" id="node_status" name="node_status" value="0" type="radio">关闭
</td>
</tr>
<tr>
<th><span class="require-red">*</span>父 节 点:</th>
<td>
<input class="common-text" type="hidden" id="node_pid" name="node_pid" size="20" value="1">
<input class="common-text" type="text" id="parent_name1" size="20" value="" readonly="true">
</td>
</tr>
<tr>
<th><span class="require-red">*</span>应用级别:</th>
<td>
<input class="common-text" type="text" id="node_level" name="node_level" size="20" value="1" readonly="true">
</td>
</tr>
<tr>
<th colspan="2">
<input type="submit"class="btn btn-primary btn6 mr10" value="添加" id="add">
<input type="reset" class="btn btn-primary btn6 mr10" value="重置">
</th>
</tr>
</tbody>
</table>
</form>
</div>
<!-- 修改菜单 Start-->
<div class="node-dlg-edit">
<form name="addFrom" id="addFrom" accept-charset="utf-8" action="<?php echo U('/Admin/Node/edit');?>" method="post">
<input type="hidden" id="edit_id" name="node_id" value="0">
<table class="admin-tab" width="450px">
<tbody>
<div class="node-dlg-title">修改节点
<div class="node-dlg-title-close"><a href="javascript:closeEditDlg();" title="关闭" >×</a></div>
</div>
<tr>
<th><span class="require-red">*</span>节点名称:</th>
<td>
<input class="common-text" type="text" id="edit_name" name="node_name" size="20" value="">
</td>
</tr>
<tr>
<th><span class="require-red">*</span>节点描述:</th>
<td>
<input class="common-text" type="text" id="edit_remark" name="node_remark" size="40" value="">
</td>
</tr>
<tr>
<th><span class="require-red">*</span>是否启用:</th>
<td>
<input class="common-text" id="edit_status" name="node_status" value="1" type="radio" checked = "checked">开启
<input class="common-text" id="edit_status" name="node_status" value="0" type="radio">关闭
</td>
</tr>
<tr>
<th><span class="require-red">*</span>父 节 点:</th>
<td>
<input class="common-text" type="hidden" id="edit_pid" name="node_pid" size="20" value="1" readonly="true">
<input class="common-text" type="text" id="parent_name2" size="20" value="" readonly="true">
</td>
</tr>
<tr>
<th><span class="require-red">*</span>应用级别:</th>
<td>
<input class="common-text" type="text" id="edit_level" name="node_level" size="20" value="1" readonly="true">
</td>
</tr>
<tr>
<th colspan="2">
<input type="submit"class="btn btn-primary btn6 mr10" value="修改" id="add">
<input type="reset" class="btn btn-primary btn6 mr10" value="重置">
</th>
</tr>
</tbody>
</table>
</form>
</div>
<!-- 弹出菜单 End -->
</body>
</html><file_sep>/Runtime/Cache/Admin/05e028d2fdcac36e7055e2e81468e3d1.php
<?php if (!defined('THINK_PATH')) exit();?><!doctype html>
<html>
<head>
<meta charset="UTF-8">
<link rel="stylesheet" type="text/css" href="/myweb/xinyi/Apps/Admin/Public/css/admin.css" />
</head>
<body>
<div class="topbar-wrap">
<div class="topbar-inner">
<div class="topbar-logo-wrap">
<h4 class="topbar-logo"><?php if($username != ''): echo ($username); else: ?>未登陆<?php endif; ?></h4>
</div>
</div>
</div>
<div class="sidebar-wrap">
<div class="sidebar-content">
<ul class="sidebar-list">
<?php if($super||in_array("/Admin/Role",$paths)||in_array("/Admin/Node",$paths)||in_array("/Admin/Access",$paths)||in_array("/Admin/Verify",$paths)){ ?>
<li><a href="#"><i class="icon-font"></i>访问权限</a>
<ul class="sub-menu">
<?php if($super||in_array("/Admin/Role",$paths)){ ?><li><a href="<?php echo U('/Admin/Role');?>"><i class="icon-font"></i>角色管理</a></li><?php } ?>
<?php if($super||in_array("/Admin/Node",$paths)){ ?><li><a href="<?php echo U('/Admin/Node');?>"><i class="icon-font"></i>节点管理</a></li><?php } ?>
<?php if($super||in_array("/Admin/Access",$paths)){ ?><li><a href="<?php echo U('/Admin/Access');?>"><i class="icon-font"></i>权限设置</a></li><?php } ?>
<?php if($super||in_array("/Admin/Verify",$paths)){ ?><li><a href="<?php echo U('/Admin/Verify');?>"><i class="icon-font"></i>申核用户</a></li><?php } ?>
</ul>
</li>
<?php } ?>
<?php if($super||in_array("/Admin/Welcome",$paths)){ ?>
<li><a href="#"><i class="icon-font"></i>移动端管理</a>
<ul class="sub-menu">
<?php if($super||in_array("/Admin/Welcome",$paths)){ ?><li><a href="<?php echo U('/Admin/Welcome');?>"><i class="icon-font"></i>欢迎界面广告</a></li><?php } ?>
<?php if($super||in_array("/Admin/HomeShop",$paths)){ ?><li><a href="<?php echo U('/Admin/HomeShop');?>"><i class="icon-font"></i>首页商家推荐</a></li><?php } ?>
<?php if($super||in_array("/Admin/Product/find",$paths)){ ?><li><a href="<?php echo U('/Admin/Product/find');?>"><i class="icon-font"></i>首页产品推荐</a></li><?php } ?>
</ul>
</li>
<?php } ?>
<?php if($super||in_array("/Admin/Attr",$paths)||in_array("/Admin/Type",$paths)||in_array("/Admin/Product/find",$paths)||in_array("/Admin/Product",$paths)){ ?>
<li><a href="#"><i class="icon-font"></i>商品管理</a>
<ul class="sub-menu">
<?php if($super||in_array("/Admin/Attr",$paths)){ ?><li><a href="<?php echo U('/Admin/Attr');?>"><i class="icon-font"></i>商品属性</a></li><?php } ?>
<?php if($super||in_array("/Admin/Type",$paths)){ ?><li><a href="<?php echo U('/Admin/Type');?>"><i class="icon-font"></i>商品分类</a></li><?php } ?>
<?php if($super||in_array("/Admin/Product/find",$paths)){ ?><li><a href="<?php echo U('/Admin/Product/find');?>"><i class="icon-font"></i>商品查询</a></li><?php } ?>
<?php if($super||in_array("/Admin/Product",$paths)){ ?><li><a href="<?php echo U('/Admin/Product');?>"><i class="icon-font"></i>添加商品</a></li><?php } ?>
</ul>
</li>
<?php } ?>
<li><a href="#"><i class="icon-font"></i>用户管理</a>
<ul class="sub-menu">
<?php if($username!=null){ ?><li><a href="#"><i class="icon-font"></i>修改资料</a></li><?php } ?>
<li><a href="<?php echo U('/Admin/Register');?>"><i class="icon-font"></i>注册商店</a></li>
<li><a href="<?php echo U('/Admin/Login/logout');?>"><?php if($username != ''): ?><i class="icon-font"></i>退出登陆<?php else: ?><i class="icon-font"></i>登陆帐号<?php endif; ?></a></li>
</ul>
</li>
</ul>
</div>
</div>
</body>
</html><file_sep>/Apps/Admin/Public/js/admin-role.js
$(document).ready(function() {
$('#add').click(function() {
if ($('#role_name').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#role_name').focus();
return false;
}
if ($('#role_remark').val() == "") {
// alert("请选择分类!");
// document.getElementById("message").innerHTML = "名称不能为空!";
$('#role_remark').focus();
return false;
}
$('#addfrom').submit();
});
});
function postdel(s1,s2,s3,s4,s5) {
$.ajax({
url: delurl,
type: 'post',
data: "id="+s1+"&name="+s2+"&pid="+s3+"&status="+s4+"&remark="+s5,
success: function(){
parent.location.reload();
window.location.reload();
},
dataType: 'html'
});
}
function poststatus(s1,s2,s3,s4,s5) {
$.ajax({
url: statusurl,
type: 'post',
data: "id="+s1+"&name="+s2+"&pid="+s3+"&status="+s4+"&remark="+s5,
success: function(){
parent.location.reload();
window.location.reload();
},
dataType: 'html'
});
}
<file_sep>/Apps/Common/Model/ProductAllModel.class.php
<?php
namespace Common\Model;
use Think\Model\RelationModel;
class ProductAllModel extends RelationModel{
//定义主表
protected $tableName = 'product';
//定义关联关系
protected $_link = array(
'imgurl' => array(
'mapping_type' => self::HAS_ONE,
'class_name' => 'product_imgurl', //要关联的模型类名
'foreign_key' => 'product_id', //关联的外键名称
// 'mapping_name' => 'articles',
'mapping_name' => 'imgurl',
'mapping_order' => 'product_id desc', //关联查询的排序
// 'mapping_fields'=> 'imageurl',
'as_fields'=>'imgurl',
),
'pattr'=>array(
'mapping_type' => self::MANY_TO_MANY,
'class_name' => 'pattr',
'foreign_key'=>'product_id',
'relation_key'=>'pattr_id',
'relation_table'=>'fz_product_pattr',
'mapping_fields'=>'name,color',
),
'ptype_name'=>array(
'mapping_type' => self::BELONGS_TO,
'class_name' => 'ptype', //要关联的模型类名
'foreign_key' => 'ptype_id', //关联的外键名称
// 'mapping_name' => 'ptype_name',
'mapping_fields'=> 'ptype_name',
'as_fields'=>'ptype_name',
),
);
}<file_sep>/Apps/API/Controller/UserController.class.php
<?php
namespace API\Controller;
use Think\Controller;
/**
* 移动端登陆验证
* @author FlyZebra
*
*/
class UserController extends Controller {
public function index() {
}
/**
* 注册用户
*/
public function register() {
if (! IS_POST) $this->error("页面不存在!");
//如果传入的参数没有帐号和密码这两个参数,直接结束。
if($_POST['loginname']==""&&$_POST['loginword']=="") die("us empty");
//导入自定义User处理类
import("Cls.User",APP_PATH);
//用户名已存在返回错误信息。
if(\User::verifyName("user", $_POST['loginname'])) die("us exist");
//向数据表插入新用户数据。
$data=\User::insertUser("user", $_POST);
if(!$data) die("注册帐号失败,请重试!");
//插入成功,更新用户登陆信息
$log=\User::insertLog("user_log", $data["id"]);
if(!$log) die("注册帐号成功,请登陆!");
// 返回包含用户信息的json字符串
\User::echoUserJson( $data, $log );
}
/**
* 验证登陆信息
*/
public function login() {
if (!IS_POST) $this->error("页面不存在!");
import("Cls.User",APP_PATH);
// 使用客户端注册用户登陆
if ($_POST ['loginname']){
$data = \User::verifyUser("user", $_POST['loginname'], $_POST['loginword']);
if($data == -1) die("no user");
if($data == 0) die("pw error");
//验证通过,更新用度等级//因为用用户登陆次数来判断用户等级
if (!(\User::upUserLevel ('user', $data))) die("更新数户数据出错,请重试!");
// 更新用户登陆信息
$log = \User::insertLog ( "user_log", $data ["id"] );
if (! $log) die( "更新用户信息出错,可能服务器连接超时,请重试!" );
\User::echoUserJson( $data, $log );
}
// 使用QQ第三方登陆,使用BAIDU第三方登陆
elseif ($_POST ['loginwith'] == "QQ" || $_POST ['loginwith'] == "BAIDU") {
// 如果已经注册返回已数据表中的已有用户信息
$data = M('user')->where ( array('openid'=>$_POST['openid']) )->find ();
// 如果QQ方式没有注册则注册新用户。这里为什么会超时?
if(!$data){
$data=\User::insertUser("user", $_POST);
if(!$data) die("注册帐号失败,请重试!");
}else {
if (!(\User::upUserLevel ('user', $data))) die("更新数户数据出错,请重试!");
}
$log = \User::insertLog ( "user_log", $data ["id"] );
if (! $log) die( "更新用户信息出错,可能服务器连接超时,请重试!" );
\User::echoUserJson( $data, $log );
}
// 使用SINA第三方登陆
elseif ($_POST ['loginwith'] == "SINA") {
}
}
/**
* 更新用户登陆信息
*/
public function upLoginInfo() {
if (! IS_POST) die ();
//导入自定义User处理类
import("Cls.User",APP_PATH);
$data = M("user")->where(array('id'=>$_POST['id']))->find();
//验证通过,更新用度等级//因为用用户登陆次数来判断用户等级
if (!(\User::upUserLevel ('user', $data))) die ("更新数户数据出错,请重试!");
// 更新用户登陆信息
$log = \User::insertLog ( "user_log", $data ["id"] );
if (! $log) die ( "更新用户信息出错,可能服务器连接超时,请重试!" );
// 返回包含用户信息的json字符串
\User::echoUserJson( $data, $log );
}
}<file_sep>/Runtime/Cache/Admin/ff5284c55541268002e4c7802b5ab64b.php
<?php if (!defined('THINK_PATH')) exit();?><!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>宇翔科技后台管理-商品管理</title>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/jquery-1.9.0.min.js"></script>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/admin-product.js"></script>
</head>
<body>
<div class="crumb-wrap">
<span class="icon-font">  <a href="<?php echo U('/Admin');?>">首页</a></span>
<span class="crumb-step">> <a href="#">添加商品</a></span>
</div>
<div class="main-wrap">
<div class="result-wrap">
<form action="<?php echo U('/Admin/Product/add');?>" method="post" id="addFrom" name="addFrom" enctype="multipart/form-data">
<table class="insert-tab" width="100%">
<tbody>
<tr>
<th width="120"><span class="require-red">*</span>商品类型:</th>
<td><select name="ptype_id" id="ptype_name" class="select_type">
<option value="">===请选择分类===</option>
<?php if(is_array($ptype)): foreach($ptype as $key=>$v): ?><option value="<?php echo ($v["id"]); ?>"><?php echo ($v["ptype_name"]); ?></option><?php endforeach; endif; ?>
</select></td>
</tr>
<tr>
<th><span class="require-red"> </span>商品属性:</th>
<td>
<div name="checkbox_css" id = "checkbox_css">
<?php if(is_array($pattr)): foreach($pattr as $key=>$v): ?><input class="checkbox1" type="checkbox" name="pattr_id[]" id="pattr_id" value="<?php echo ($v["id"]); ?>">
<span style="background:<?php echo ($v["color"]); ?>;color:#FFFFFF">[<?php echo ($v["name"]); ?>]</span> <?php endforeach; endif; ?>
</div>
</td>
</tr>
<tr>
<th><span class="require-red">*</span>商品名称:</th>
<td><input class="common-text" id="name" name="name"
size="50" value="" type="text"></td>
</tr>
<tr>
<th><span class="require-red">*</span>商品价格:</th>
<td><input class="common-text" id="price" name="price"
size="15" value="" type="text">元</td>
</tr>
<tr>
<th><span class="require-red">*</span>商品图像1:</th>
<td><input class="common-text" name="proimg1" id="proimg1"
type="file" accept=".jpg,.png"><span class="require-red">支持格式PNG、JPG,分辨率:480*320。</span> <!--<input class="btn btn6" type="submit" onclick="submitForm('/jscss/admin/design/upload')" value="上传图片"/>-->
</td>
</tr>
<tr>
<th><span class="require-red"> </span>商品图像2:</th>
<td><input class="common-text" name="proimg2" id="proimg2"
type="file" accept=".jpg,.png"><span class="require-red">支持格式PNG、JPG,分辨率:480*320。</span> <!--<input type="submit" onclick="submitForm('/jscss/admin/design/upload')" value="上传图片"/>-->
</td>
</tr>
<tr>
<th><span class="require-red"> </span>商品图像3:</th>
<td><input class="common-text" name="proimg3" id="proimg3"
type="file" accept=".jpg,.png"><span class="require-red">支持格式PNG、JPG,分辨率:480*320。</span> <!--<input type="submit" onclick="submitForm('/jscss/admin/design/upload')" value="上传图片"/>--></td>
</tr>
<tr>
<th><span class="require-red"> </span>商品图像4:</th>
<td><input class="common-text" name="proimg4" id="proimg4"
type="file" accept=".jpg,.png"><span class="require-red">支持格式PNG、JPG,分辨率:480*320。</span> <!--<input type="submit" onclick="submitForm('/jscss/admin/design/upload')" value="上传图片"/>--></td>
</tr>
<tr>
<th><span class="require-red"> </span>商品图像5:</th>
<td><input class="common-text" name="proimg5" id="proimg5"
type="file" accept=".jpg,.png"><span class="require-red">支持格式PNG、JPG,分辨率:480*320。</span> <!--<input type="submit" onclick="submitForm('/jscss/admin/design/upload')" value="上传图片"/>--></td>
</tr>
<tr>
<th><span class="require-red">*</span>商品描述:</th>
<td><textarea name="describe" class="common-textarea"
id="describe" cols="30" style="width: 98%;" rows="10"></textarea></td>
</tr>
<tr>
<th></th>
<td><input type="submit" class="btn btn-primary btn6 mr10"
value="添加" id="addproduct"> <input type="reset"
class="btn btn-primary btn6 mr10" value="重置"></td>
</tr>
</tbody>
</table>
<input type="hidden" name="shopname" value="<?php echo ($shopname); ?>">
<input type="hidden" name="shop_id" value="<?php echo ($shop_id); ?>">
</form>
</div>
</div>
</body>
</html><file_sep>/Runtime/Cache/Admin/9863cdbd814baf9e9ab93473c90cfc4b.php
<?php if (!defined('THINK_PATH')) exit();?><!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>宇翔科技登陆界面</title>
<link rel="stylesheet" type="text/css" href="/myweb/xinyi/Apps/Admin/Public/css/login.css" />
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/jquery-1.9.0.min.js"></script>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/md5.js"></script>
<script type="text/javascript"> var ajaxurl= '<?php echo U("/Admin/Ajax/log");?>'</script>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/admin-login.js"></script>
</head>
<body>
<div class="login-mask"></div>
<div class="login-center">
<form name="loginform" id="loginform" accept-charset="utf-8" action="<?php echo U('/Admin/Login/login');?>" method="post">
<table class="login-tab" width=100%>
<tbody>
<tr>
<th colspan="2">管理员登陆</th>
</tr>
<tr>
<td style="width:35%;text-align:right">帐号:</td>
<td><input class="common-text" id="shopname" name="shopname" size="20" value="" type="text"></td>
</tr>
<tr>
<td style="text-align:right">密码:</td>
<td><input class="common-text" id="password" name="password" size="20" value="" type="password"></td>
</tr>
<tr>
<th colspan="2" >
<input type="button" class="btn btn-primary btn100" value="登陆" id="login">
<input type="reset" class="btn btn-primary btn100" value="重置"></th>
</tr>
<tr>
<td style="text-align:center" colspan="2">
<div id="message"></div>
</td>
</tr>
</tbody>
</table>
</form>
</div>
</body>
</html><file_sep>/Apps/API/Conf/config.php
<?php
return array(
//'配置项'=>'配置值'
'URL_ROUTER_ON' => true, // 开启路由
'URL_ROUTE_RULES'=>array(
'Product/id/:id'=>'API/Product/id',
'Product/Type/:type'=>'API/Product/type',
),
);<file_sep>/Apps/Admin/Public/js/admin-verify.js
function verify(s1) {
openDlg();
var s2 = $("#role"+s1).val();
$.ajax({
url: ajaxurl,
type: 'post',
data: "user_id="+s1+"&role_id="+s2,
success: function(result) {
parent.location.reload();
window.location.reload();
},
dataType: 'html'
});
}
function openDlg() {
$('.dlg-content').fadeIn(100);
$('.dlg-mask').fadeIn(100);
}
function closeDlg() {
$('.dlg-content').fadeOut(100);
$('.dlg-mask').fadeOut(100);
}
<file_sep>/Runtime/Cache/Admin/ebb58a319a155968b09ce38983312dae.php
<?php if (!defined('THINK_PATH')) exit();?>
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>宇翔科技后台管理</title>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/jquery-1.9.0.min.js"></script>
<script type="text/javascript">
var ajaxurl = '<?php echo U("/Admin/Verify/verify");?>'
</script>
<script type="text/javascript" src="/myweb/xinyi/Apps/Admin/Public/js/admin-verify.js"></script>
</head>
<body>
<div class="crumb-wrap">
<span class="icon-font">  <a href="<?php echo U('/Admin');?>">首页</a></span>
<span class="crumb-step">> <a href="#">申核用户</a></span>
</div>
<div class="main-wrap">
<div class="result-wrap">
<table class="admin-tab" width="100%">
<tbody align="right">
<tr style="background: #efefef">
<th width="5%">ID</th>
<th width="10%">商店名称</th>
<th width="8%">店主姓名</th>
<th width="10%">店主手机</th>
<th width="10%">商店电话</th>
<th>商店地址</th>
<th width="14%">注册时间</th>
<th width="15%">申核操作</th>
</tr>
<?php if(is_array($shop)): foreach($shop as $key=>$s): ?><tr>
<th><?php echo ($s["id"]); ?></th>
<td><?php echo ($s["shopname"]); ?></td>
<td><?php echo ($s["peoplename"]); ?></td>
<th><?php echo ($s["peoplephone"]); ?></th>
<th><?php echo ($s["shopphone"]); ?></th>
<td><?php echo ($s["address"]); ?></td>
<th><?php echo (date("Y-m-d H:i",$s["regtime"])); ?></th>
<th><select style="padding-bottom: 5px; height: 26px; font-size: 14px" name="role<?php echo ($s["id"]); ?>" id="role<?php echo ($s["id"]); ?>">
<option>未申核</option>
<?php if(is_array($role)): foreach($role as $key=>$r): if($r['id'] == $s['role_id']): ?><option value="<?php echo ($r["id"]); ?>" selected="selected"><?php echo ($r["remark"]); ?></option>
<?php else: ?>
<option value="<?php echo ($r["id"]); ?>"><?php echo ($r["remark"]); ?></option><?php endif; endforeach; endif; ?>
</select><input type="button" class="btn btn-primary" value="通过申核" onclick="javascript:verify('<?php echo ($s["id"]); ?>');">
</th>
</tr><?php endforeach; endif; ?>
</tbody>
</table>
</div>
</div>
<div class="dlg-mask"></div>
<!-- 添加菜单 Start-->
<div class="dlg-content">正在向服务器提交请求.....</div>
</body>
</html> | 9396f1f02037566afafe1c83e4112eb4b2c49ee6 | [
"JavaScript",
"SQL",
"PHP"
] | 52 | PHP | TTFlyzebra/phpxinyi | d4cef8a385136da991f2d337bb58d35b69446741 | 15e4bb7fe88f6c8333695a042a03e05cd7d4b24f | |
refs/heads/main | <repo_name>DenisSlipko/GTSPA<file_sep>/src/components/Map.jsx
function Map() {
return (
<div className="map-container">
<p className="map_title">Где нас найти ?</p>
<iframe
title="map"
className="g-map"
style={{
frameBorder: "0",
scrolling: "no",
marginHeight: "0",
marginWidth: "0",
}}
src="https://maps.google.com/maps?width=100%25&height=400&hl=en&q=dnipro,%20lyvarna%20sr%204+(Your%20Business%20Name)&t=p&z=17&ie=UTF8&iwloc=B&output=embed"
></iframe>
</div>
);
}
export default Map;
<file_sep>/src/components/Offer.jsx
function Offer() {
return (
<div className="offer-container">
<p className="offer-title">ЗАЯВКА НА ПОДБОР ТУРА</p>
<p className="offer-subtitle">
Заполните заявку и наш менеджер свяжется с вами и подберет идеальные
условия для вашего путешествия
</p>
<div className="input-container">
<div className="first-input-wrapper">
<input type="text" placeholder="Ваше имя" className="name-input" />
<input
type="text"
placeholder="Ваш телефон"
className="phone-input"
/>
</div>
<textarea
type="text"
placeholder="Ваш комментарий"
className="coment-input"
/>
</div>
<div className="btn-offer-wrapper">
<button className="btn-offer-send">Отправить</button>
<p className="subtitle-btn">
Нажимая на кнопку отправить вы соглашаетесь с условиями политики
конфиденциальности и обработки персональных данных
</p>
</div>
</div>
);
}
export default Offer;
<file_sep>/src/components/WhyUs.jsx
import svgMap from "../img/svgMap.svg";
export default function WhyUs() {
return (
<div className="whyus-container">
<div className="w-container">
<p className="w-title">ПОЧЕМУ СТОИТ ВЫБРАТЬ НАС?</p>
<p className="w-text light">
Мы работаем с людьми, а не с турами.
<br />
Самое важное для нас решить задачи клиента
</p>
<div className="w-list">
<div className="col-item">
<div className="w-item">
<img src="" alt="" className="w-logo" />
<p className="w-item-text">Опыт в подборе туров чёто типа</p>
</div>
<div className="w-item">
<img src="" alt="" className="w-logo" />
<p className="w-item-text">Время на рынке</p>
</div>
</div>
<div className="col-item">
<div className="w-item">
<img src="" alt="" className="w-logo" />
<p className="w-item-text">
Сопровождение и отзывчивость во время отдыха
</p>
</div>
<div className="w-item">
<img src="../img/.jpg" alt="" className="w-logo" />
<p className="w-item-text">Хорошие цены</p>
</div>
</div>
</div>
<div className="btn-container">
<button className="btn header about">Выбрать тур</button>
<button className="btn header w about">Выбрать тур</button>
</div>
</div>
<img
src={svgMap}
alt="map"
style={{
textAlign: "end",
position: "absolute",
opacity: "0.3",
right: 0,
marginTop: "100px",
}}
/>
</div>
);
}
<file_sep>/src/components/Carousel.jsx
import React, { Component } from "react";
import Slider from "react-slick";
import "./style.css";
import img from "../img/bgimg.jpg";
export default class MultipleItems extends Component {
render() {
const settings = {
dots: false,
infinite: true,
speed: 500,
slidesToShow: 3,
slidesToScroll: 1,
initialSlide: 0,
responsive: [
{
breakpoint: 925,
settings: {
slidesToShow: 2,
slidesToScroll: 1,
infinite: true,
dots: true,
},
},
{
breakpoint: 768,
settings: {
slidesToShow: 2,
slidesToScroll: 1,
initialSlide: 2,
},
},
{
breakpoint: 375,
settings: {
slidesToShow: 1,
slidesToScroll: 1,
dots: true,
},
},
],
};
const obj = [
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
title: "Каникулы в Египте",
img: `${img}`,
},
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
title: "Каникулы в Египте",
img: `${img}`,
},
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
title: "Каникулы в Египте",
img: `${img}`,
},
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
title: "Каникулы в Египте",
img: `${img}`,
},
];
return (
<div className="slider-container">
<h2 style={{ textTransform: "uppercase", marginBottom: "60px" }}>
{" "}
Актуальные туры{" "}
</h2>
<Slider {...settings}>
{obj.map((item) => (
<div className="card-container">
<div className="card-img">
<img src={item.img} alt="" className="image" />
</div>
<p className="carousel-title">{item.title}</p>
<p className="carousel-text">{item.text}</p>
</div>
))}
</Slider>
</div>
);
}
}
<file_sep>/src/layout/Header.jsx
import { Link } from "react-router-dom";
import tgiconW from "../icons/tgiconW.svg";
function Header() {
const handleColorClick = () => {
document.querySelector(".brand-text").style = "color: #595959";
document.querySelector(".brand-text-small").style = "color: #595959";
document
.querySelectorAll(".nav-link")
.forEach((el) => (el.style = "color: #595959"));
document.querySelector(".header-phone").style = "color: #595959";
};
const handleReverseColor = () => {
document.querySelector(".brand-text").style = "color: white";
document.querySelector(".brand-text-small").style = "color: white";
document
.querySelectorAll(".nav-link")
.forEach((el) => (el.style = "color: white"));
document.querySelector(".header-phone").style = "color: white";
};
const handleMenuClick = () => {
let element = document.querySelector(".navbar-toggler");
element.classList.toggle("open");
};
return (
<nav className="navbar navbar-expand-lg navbar-light bg-light">
<div className="container-fluid">
<Link
className="navbar-brand"
to="/"
onClick={() => handleReverseColor()}
>
<div className="brand-container">
<div className="brand-text"><NAME></div>
<p className="brand-text-small">туристическая фирма</p>
</div>
</Link>
<button
className="navbar-toggler"
type="button"
data-bs-toggle="collapse"
data-bs-target="#navbarNavDropdown"
aria-controls="navbarNavDropdown"
aria-expanded="false"
aria-label="Toggle navigation"
onClick={() => handleMenuClick()}
>
<span className="navbar-toggler-icon"></span>
</button>
<div className="collapse navbar-collapse" id="navbarNavDropdown">
<ul className="navbar-nav ml-auto">
<li className="nav-item">
<Link
className="nav-link"
aria-current="page"
to="/"
onClick={() => handleReverseColor()}
>
Главная
</Link>
</li>
<li className="nav-item">
<Link
className="nav-link"
to="/about"
onClick={() => handleColorClick()}
>
О нас
</Link>
</li>
<li className="nav-item">
<Link
className="nav-link"
to="/places"
onClick={() => handleColorClick()}
>
Страны
</Link>
</li>
<li className="nav-item last">
<Link
className="nav-link"
to="/offer"
onClick={() => handleColorClick()}
>
Оставить запрос
</Link>
</li>
</ul>
<a href="#!" className="tg-icon-link">
<img className="header-icon-tg" src={tgiconW} alt="telegram" />
</a>
<div className="white-space-container"></div>
<p className="header-phone">+38 (067) 749-80-55</p>
<button className="btn header">Перезвонить мне</button>
<p className="location-header">
Украина, Днепр,
<br /> ул. Литейная 4
</p>
</div>
</div>
</nav>
);
}
export default Header;
<file_sep>/src/components/Card.jsx
import { useState } from "react";
import img from "../img/background.jpg";
import img2 from "../img/backgroundHP.jpg";
import ModalWindow from "./ModalWindow";
function Card() {
const obj = [
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
full_text: `Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных. \n Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных.Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных. Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных`,
title: "Каникулы в Египте",
imgBig: `${img}`,
imgSmall1: `${img}`,
imgSmall2: `${img2}`,
imgSmall3: `${img2}`,
imgSmall4: `${img2}`,
},
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
full_text:
"Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных. Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных.Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных. Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных",
title: "Каникулы в Египте",
imgBig: `${img}`,
},
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
full_text: `Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных.\n Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных. \n Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных. Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных`,
title: "Каникулы в Египте",
imgBig: `${img}`,
},
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
title: "Каникулы в Египте",
imgBig: `${img}`,
},
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
full_text:
"Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных. Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных.Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных. Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных",
title: "Каникулы в Египте",
imgBig: `${img}`,
},
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
full_text:
"Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных. Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных.Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных. Нажимая на кнопку отправить вы соглашаетесь с условиями политики конфиденциальности и обработки персональных данных",
title: "Каникулы в Египте",
imgBig: `${img}`,
},
];
const [modalActive, setModalActive] = useState(false);
const [modalText, setModalText] = useState("");
const [modalTitle, setModalTitle] = useState("");
const [modalBigImg, setModalBigImg] = useState("");
const [modalFullText, setModalFullText] = useState("");
const [modalImg1, setModalImg1] = useState("");
const [modalImg2, setModalImg2] = useState("");
const [modalImg3, setModalImg3] = useState("");
const [modalImg4, setModalImg4] = useState("");
return (
<>
<div className="card-container">
<p className="card-text">Популярные Направления</p>
<div className="card-wrapper">
{obj.map((item) => (
<>
<div
className="card"
onClick={() => {
setModalActive(true);
setModalText(item.text);
setModalTitle(item.title);
setModalBigImg(item.imgBig);
setModalFullText(item.full_text);
setModalImg1(item.imgSmall1);
setModalImg2(item.imgSmall2);
setModalImg3(item.imgSmall3);
setModalImg4(item.imgSmall4);
}}
>
<div className="card-text-container">
<img src={item.imgBig} alt="" className="card-img" />
<p className="card-title">{item.title}</p>
</div>
<p className="card-subtitle">{item.text}</p>
</div>
</>
))}
</div>
</div>
<ModalWindow
active={modalActive}
setActive={setModalActive}
text={modalText}
title={modalTitle}
imgBig={modalBigImg}
fullText={modalFullText}
imgSmall1={modalImg1}
imgSmall2={modalImg2}
imgSmall3={modalImg3}
imgSmall4={modalImg4}
/>
</>
);
}
export default Card;
<file_sep>/src/pages/About.jsx
import ComentCarousel from "../components/ComentCarousel";
import Map from "../components/Map";
function About() {
return (
<>
<ComentCarousel />
<Map />
</>
);
}
export default About;
<file_sep>/src/pages/Homepage.jsx
import Carousel from "../components/Carousel";
import "./style.css";
import Card from "../components/Card";
import WhyUs from "../components/WhyUs";
import Offer from "../components/Offer";
import ComentCarousel from "../components/ComentCarousel";
import Map from "../components/Map";
function Homepage() {
return (
<>
<div className="home-container">
<div className="text">
<p className="pre-title">Незабываемые туры в разные страны</p>
<p className="main-title">ГРАНД ТУР</p>
<p className="post-title">
Хотите посетить новые уголки мира и оставить яркие впечатления?{" "}
<br /> Тогда вы по адресу!
</p>
<button className="btn main" style={{ marginTop: "50px" }}>
Посмотреть предложения
</button>
</div>
</div>
<div className="carousel-container">
<Carousel />
</div>
<WhyUs />
<Card />
<Offer />
<ComentCarousel />
<Map />
</>
);
}
export default Homepage;
<file_sep>/src/components/ComentCarousel.jsx
import React, { Component } from "react";
import Slider from "react-slick";
import "./style.css";
import img from "../img/bgimg.jpg";
import tgicon from "../icons/tgicon.svg";
export default class ComentCarousel extends Component {
render() {
const settings = {
dots: true,
infinite: true,
speed: 500,
slidesToShow: 1,
slidesToScroll: 1,
initialSlide: 0,
responsive: [
{
breakpoint: 925,
settings: {
slidesToShow: 1,
slidesToScroll: 1,
infinite: true,
dots: true,
},
},
{
breakpoint: 768,
settings: {
slidesToShow: 1,
slidesToScroll: 1,
initialSlide: 2,
},
},
{
breakpoint: 375,
settings: {
slidesToShow: 1,
slidesToScroll: 1,
dots: true,
},
},
],
};
const obj = [
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
personName: "<NAME>",
img: `${img}`,
tgIcon: `${tgicon}`,
instIcon: `${tgicon}`,
},
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
personName: "<NAME>",
img: `${img}`,
tgIcon: `${tgicon}`,
instIcon: `${tgicon}`,
},
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
personName: "<NAME>",
img: `${img}`,
tgIcon: `${tgicon}`,
instIcon: `${tgicon}`,
},
{
text:
"Вкус рождества в окружении чудесных пирамид, вы такое пробовали? Очутиться в жаркой стране посреди зимы - это то, что нужно!",
personName: "<NAME>",
img: `${img}`,
tgIcon: `${tgicon}`,
instIcon: `${tgicon}`,
},
];
return (
<div className="coment-slider">
<Slider {...settings} style={{ width: "40%" }}>
{obj.map((item) => (
<div className="coment-container">
<div className="info-coment">
<img src={img} alt="" className="person-photo" />
<div className="info-person">
<p className="person-name">{item.personName}</p>
<div className="ntw-container">
<a href="#!">
<img
className="tg-icon"
src={item.tgIcon}
alt="telegram"
/>
</a>
<a href="#!">
<img
className="inst-icon"
src={item.instIcon}
alt="telegram"
/>
</a>
</div>
</div>
</div>
<div className="coment-text">{item.text}</div>
</div>
))}
</Slider>
</div>
);
}
}
<file_sep>/src/App.js
import { useTransition, animated } from "react-spring";
import { Route, Switch, useLocation } from "react-router-dom";
import Header from "./layout/Header";
import Footer from "./layout/Footer";
import About from "./pages/About";
import AboutPlaces from "./pages/AboutPlaces";
import Homepage from "./pages/Homepage";
import Offer from "./pages/Offer";
function App() {
const location = useLocation();
const transitions = useTransition(location, (location) => location.pathname, {
from: { opacity: 0 },
enter: { opacity: 1 },
leave: { opacity: 0 },
});
return (
<>
<Header />
<main className="main-container">
{transitions.map(({ item, props, key }) => (
<animated.div key={key} style={props}>
<div style={{ position: "absolute", width: "100%" }}>
<Switch location={item}>
<Route exact path="/" component={Homepage} />
<Route exact path="/about" component={About} />
<Route exact path="/places" component={AboutPlaces} />
<Route exact path="/offer" component={Offer} />
</Switch>
</div>
</animated.div>
))}
</main>
<Footer />
</>
);
}
export default App;
<file_sep>/src/components/ModalWindow.jsx
import React from "react";
import "./style.css";
const ChangeImg = (str) => {
document.getElementById("modal__img__id").setAttribute("src", str);
};
const ModalWindow = ({
active,
setActive,
fullText,
imgBig,
imgSmall1,
imgSmall2,
imgSmall3,
imgSmall4,
title,
}) => {
return (
<div
className={active ? "modal_w active" : "modal_w"}
onClick={() => setActive(false)}
style={{ padding: "20px 200px" }}
>
<div
className={active ? "modal__content active" : "modal__content"}
onClick={(e) => e.stopPropagation()}
>
<button
onClick={() => setActive(false)}
className="btn_exit_modal"
></button>
<div className="img__container">
<div className="small__img__container">
<img
src={imgSmall1}
alt=""
className="small__modal__img"
onClick={() => ChangeImg(imgSmall1)}
/>
<img
src={imgSmall2}
alt=""
className="small__modal__img"
onClick={() => ChangeImg(imgSmall2)}
/>
<img
src={imgSmall3}
alt=""
className="small__modal__img"
onClick={() => ChangeImg(imgSmall3)}
/>
<img
src={imgSmall4}
alt=""
className="small__modal__img"
onClick={() => ChangeImg(imgSmall4)}
/>
</div>
<img
src={imgBig}
alt=""
style={{ width: "70%", marginBottom: "12px" }}
className="modal__img"
id="modal__img__id"
/>
</div>
<div className="modal__text__container">
<p className="modal__title">{title}</p>
<p className="modal__text">{fullText}</p>
</div>
</div>
</div>
);
};
export default ModalWindow;
| 7004a475387b0ef6649c3f262109d44d19030360 | [
"JavaScript"
] | 11 | JavaScript | DenisSlipko/GTSPA | 93d4273f28b03b84c28294372e4fe98d6ee57a45 | 5b606c41890212855e362f4b91f2def8a91d334b |