branch_name
stringclasses 149
values | text
stringlengths 23
89.3M
| directory_id
stringlengths 40
40
| languages
sequencelengths 1
19
| num_files
int64 1
11.8k
| repo_language
stringclasses 38
values | repo_name
stringlengths 6
114
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
|
---|---|---|---|---|---|---|---|---|
refs/heads/main | <repo_name>ReinisV/PuppeteerTaskDeadlockRepro<file_sep>/PuppeteerTaskDeadlockRepro/Program.cs
using PuppeteerSharp;
using System;
using System.Threading;
using System.Threading.Tasks;
namespace PuppeteerTaskDeadlockRepro
{
class Program
{
static ManualResetEvent quitEvent = new ManualResetEvent(false);
static ManualResetEvent browserSetEvent = new ManualResetEvent(false);
static async Task Main(string[] args)
{
var browserFetcher = new BrowserFetcher();
Console.WriteLine("fetching browser");
await browserFetcher.DownloadAsync(BrowserFetcher.DefaultRevision);
Console.WriteLine("launching browser");
// case #1: browser from current thread will deadlock on the Tasks it returns when the
// current thread is blocked by "quitEvent.WaitOne()"
// in this example, it will happen here: "await browser.NewPageAsync()"
var browser = await GetBrowserFromCurrentThread();
// case #2: if you create the browser on a different thread (that won't be blocked),
// the app will work as expected (a new tab will be opened and navigated to google every 5 seconds)
// var browser = GetBrowserFromAnotherThread();
Console.CancelKeyPress += (sender, eArgs) =>
{
Console.WriteLine("App killed");
quitEvent.Set();
eArgs.Cancel = true;
};
RunIndefinitely(browser);
quitEvent.WaitOne();
// cleanup code here
}
private static Browser GetBrowserFromAnotherThread()
{
Browser browser = null;
var thread = new Thread(async () =>
{
browser = await Puppeteer.LaunchAsync
(
new LaunchOptions
{
Headless = false
}
);
browserSetEvent.Set();
});
thread.Start();
browserSetEvent.WaitOne();
return browser;
}
private static async Task<Browser> GetBrowserFromCurrentThread()
{
return await Puppeteer.LaunchAsync
(
new LaunchOptions
{
Headless = false
}
);
}
private static void RunIndefinitely(Browser browser)
{
var thread = new Thread(async () =>
{
while (true)
{
await PrintPageInfo(browser);
Console.WriteLine("loop finished");
await Task.Delay(TimeSpan.FromSeconds(5));
}
});
thread.Start();
}
private static async Task PrintPageInfo(Browser browser)
{
var url = "https://www.google.com";
Console.WriteLine("opening new page");
var page = await browser.NewPageAsync();
Console.WriteLine("starting navigation");
await page.GoToAsync(url);
Console.WriteLine("waiting for navigation");
var contents = await page.GetContentAsync();
Console.WriteLine($"{url} : {contents.Length}");
}
}
}
<file_sep>/README.md
# PuppeteerTaskDeadlockRepro
reproduction of a bug found in Puppeteer-CSharp library
| 24580fa52855f41e233091eac9f6e3c826bbac00 | [
"Markdown",
"C#"
] | 2 | C# | ReinisV/PuppeteerTaskDeadlockRepro | 852b76682bdc81775fcf4e792f630f34e0e4e50b | 92b92dc54939d7a0bcbe966ee4d42d0d4880492d |
refs/heads/master | <file_sep>package com.example.upgraddemo.Home.model;
import android.app.Application;
import android.arch.lifecycle.LiveData;
import android.arch.lifecycle.MutableLiveData;
import android.util.Log;
import com.example.upgraddemo.Home.model.data.hot.Item;
import com.example.upgraddemo.Home.model.data.hot.QuestionsList;
import com.example.upgraddemo.Home.model.service.DataService;
import com.example.upgraddemo.Home.model.service.RetrofitClientInstance;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
public class home_repository {
// private final hotItemDao hotDao;
//
// public home_repository(Application application) {
//
// hotItemDatabase hotDatabase = hotItemDatabase.getInstance(application);
// hotDao = hotDatabase.hotDao();
// }
public LiveData<List<Item>> getHotQuestions(String Tag){
final MutableLiveData<List<Item>> allhotquestions = new MutableLiveData<>();
DataService service = RetrofitClientInstance.getRetrofitInstance().create(DataService.class);
Map<String, String> paramsMap = new HashMap<String, String>();
paramsMap.put("sort", "hot");
paramsMap.put("site", "stackoverflow");
paramsMap.put("pagesize","100");
paramsMap.put("tagged",Tag);
paramsMap.put("order","desc");
Call<QuestionsList> call = service.getHotItems(paramsMap);
call.enqueue(new Callback<QuestionsList>() {
@Override
public void onResponse(Call<QuestionsList> call, Response<QuestionsList> response) {
if(response.isSuccessful()){
allhotquestions.setValue(response.body().getItems());
}
Log.d("code","code: "+response.code());
}
@Override
public void onFailure(Call<QuestionsList> call, Throwable t) {
Log.d("Error","Error Occurred");
}
});
return allhotquestions;
}
public LiveData<List<com.example.upgraddemo.Home.model.data.week.Item>> getWeekQuestions(String Tag){
final MutableLiveData<List<com.example.upgraddemo.Home.model.data.week.Item>> allWeekquestions = new MutableLiveData<>();
DataService service = RetrofitClientInstance.getRetrofitInstance().create(DataService.class);
Map<String, String> paramsMap = new HashMap<String, String>();
paramsMap.put("sort", "week");
paramsMap.put("site", "stackoverflow");
paramsMap.put("tagged",Tag);
paramsMap.put("pagesize","100");
paramsMap.put("order","desc");
Call<com.example.upgraddemo.Home.model.data.week.QuestionsList> call = service.getWeekItems(paramsMap);
call.enqueue(new Callback<com.example.upgraddemo.Home.model.data.week.QuestionsList>() {
@Override
public void onResponse(Call<com.example.upgraddemo.Home.model.data.week.QuestionsList> call, Response<com.example.upgraddemo.Home.model.data.week.QuestionsList> response) {
if(response.isSuccessful()){
allWeekquestions.setValue(response.body().getItems());
}
Log.d("code","code: "+response.code());
}
@Override
public void onFailure(Call<com.example.upgraddemo.Home.model.data.week.QuestionsList> call, Throwable t) {
Log.d("Error","Error Occurred");
}
});
return allWeekquestions;
}
}
<file_sep>package com.example.upgraddemo.userInterest.model;
import android.arch.lifecycle.LiveData;
import android.arch.lifecycle.MutableLiveData;
import android.arch.lifecycle.ViewModel;
import android.util.Log;
import com.example.upgraddemo.userInterest.model.data.Item;
import java.util.List;
public class interest_viewmodel extends ViewModel {
private LiveData<List<Item>> Tags;
public void init(){
if (Tags == null) {
Tags = new MutableLiveData<List<Item>>();
loadTags();
}
}
public LiveData<List<Item>> getTags() {
return this.Tags;
}
private void loadTags() {
Tags = new interest_repository().getAllTags();
}
public interest_viewmodel() {
}
}
<file_sep>package com.example.upgraddemo.Home.model.data.week;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
public class MigratedFrom {
@SerializedName("other_site")
@Expose
private OtherSite otherSite;
@SerializedName("on_date")
@Expose
private Integer onDate;
@SerializedName("question_id")
@Expose
private Integer questionId;
public OtherSite getOtherSite() {
return otherSite;
}
public void setOtherSite(OtherSite otherSite) {
this.otherSite = otherSite;
}
public Integer getOnDate() {
return onDate;
}
public void setOnDate(Integer onDate) {
this.onDate = onDate;
}
public Integer getQuestionId() {
return questionId;
}
public void setQuestionId(Integer questionId) {
this.questionId = questionId;
}
}<file_sep>package com.example.upgraddemo.Home.adapter;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentStatePagerAdapter;
import com.example.upgraddemo.Home.ui.TabFragment;
public class PagerAdapter extends FragmentStatePagerAdapter {
int mNumOfTabs;
String tag;
public PagerAdapter(FragmentManager fm, int NumOfTabs, String tag) {
super(fm);
this.mNumOfTabs = NumOfTabs;
this.tag = tag;
}
@Override
public Fragment getItem(int position) {
switch (position) {
case 0:
TabFragment tab = TabFragment.newInstance(tag,"hot");
return tab;
case 1:
tab = TabFragment.newInstance(tag,"week");
return tab;
default:
return TabFragment.newInstance(tag, "hot");
}
}
@Override
public int getCount() {
return mNumOfTabs;
}
}<file_sep>package com.example.upgraddemo.userInterest.model.service;
import com.example.upgraddemo.userInterest.model.data.Item;
import com.example.upgraddemo.userInterest.model.data.TagsList;
import java.util.List;
import retrofit2.Call;
import retrofit2.http.GET;
public interface DataService {
@GET("2.2/tags?pagesize=100&order=desc&sort=popular&site=stackoverflow")
Call<TagsList> getItems();
}
| c2d455770f2d94c56e6daaa9b92c0bf4fc788eda | [
"Java"
] | 5 | Java | Cool-fire/UpgradDemo | ee8f795e79ede5830928689287fc24f7964dc846 | 96f4db8f2ff36e25d795941324bd8d45ff3d4a7c |
refs/heads/main | <file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Database extends Model
{
public $timestamps = false;
public $table = 'plants';
public function getTimeIfLateCalculateAttribute () {
return ceil((strtotime('now') - $this->last_watered - $this->days_until_water ) / 86400)-1;
}
public function getTimeCalculateAttribute() {
return ceil((($this->last_watered + $this->days_until_water) - strtotime('now')) / 86400);
}
}
<file_sep><?php
namespace App\Http\Controllers;
use App\Models\Database;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Redirect;
use Symfony\Component\Console\Input\Input;
use Symfony\Component\VarDumper\Cloner\Data;
class DatabaseController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$databases = Database::all()->sortByDesc('TimeIfLateCalculate'); // gebruikt functie uit model
return view('database.index', ['databases'=>$databases]);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
$database = Database::all();
return view('database.create', ['database'=>optional($database)]);
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$database = new Database;
$database->plant_name = $request->plant_name;
$database->water = $request->water;
$database->propogation_method = $request->propogation_method;
$database->light = $request->light;
$database->days_until_water = $request->days_until_water;
$database->last_watered = strtotime('now');
$database->save();
return Redirect::to('database/');
// return view('database.create');
}
/**
* Display the specified resource.
*
* @param \App\Models\Database $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
$database = Database::find($id);
return view('database.show', ['database' => $database]);
}
/**
* Show the form for editing the specified resource.
*
* @param \App\Models\Database $database
* @return \Illuminate\Http\Response
*/
public function edit(Database $database, $request)
{
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param \App\Models\Database $database
* @return \Illuminate\Http\Response
*/
public function update(Request $request, Database $database)
{
$database->plant_name = $request->plant_name;
$database->water = $request->water;
$database->propogation_method = $request->propogation_method;
$database->light = $request->light;
$database->days_until_water = $request->days_until_water;
$database->save();
return Redirect::to('database/{id}');
}
/**
* Remove the specified resource from storage.
*
* @param \App\Models\Database $database
* @return \Illuminate\Http\Response
*/
public function destroy(Database $database)
{
//
}
public function updateTime(Database $database)
{
$database->last_watered = strtotime('now');
$database->save();
return Redirect::to('database/');
}
}
<file_sep><?php
use App\Http\Controllers\DatabaseController;
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('layout');
});
Route::get('/todo', function () {
return view('todo.todo');
});
// Route::get('/name/{id}', [DatabaseController::class, 'show', function($id) {
// return view('database.show');
// }]);
Route::resource('database', DatabaseController::class);
Route::put('database/update/{database}', [DatabaseController::class, 'updateTime'])->name('database.update-time');
| 4abeeeb96382ec861d7dd1e285e5bf0dcbc0f653 | [
"PHP"
] | 3 | PHP | Florianbracke/Pages | c5e9a6ddb6f229ba8c3c718518e3ffd71e53d37c | 6537755380dfffa4e8c79e1b49d92fdff803856d |
refs/heads/master | <file_sep># -*- coding: utf-8 -*-
"""
Created on Wed May 17 16:06:30 2017
@author: sapjz
"""
import subprocess
import os
print(os.getcwd())
#subprocess.call('pip install --upgrade --no-deps --force-reinstall kabuki',cwd='../kabuki')
#subprocess.call('pip install --upgrade --no-deps --force-reinstall hddm',cwd='../hddm')
subprocess.call('python setup.py install',cwd='../kabuki')
subprocess.call('python setup.py install',cwd='../hddm')
<file_sep># -*- coding: utf-8 -*-
"""
Created on Wed May 17 16:57:37 2017
Adapted form the hddm tutorial http://ski.clps.brown.edu/hddm_docs/tutorial_regression_stimcoding.html
Assume a 2x2 within-subject design
Go/No-go and Session1/Session2
@author: sapjz
"""
import hddm
from patsy import dmatrix # for generation of (regression) design matrices
import numpy as np # for basic matrix operations
from pandas import Series # to manipulate data-frames generated by hddm
n_subjects = 5
trials_per_level = 150 # and per stimulus
# session 1
level1a = {'v':.3, 'a':2, 't':.3, 'sv':0, 'z':.6, 'sz':0, 'st':0}
# level2 is the nogo condition, and therefore the v is negative here (towards the no-go boundary)
level2a = {'v':-1, 'a':2, 't':.3, 'sv':0, 'z':.4, 'sz':0, 'st':0}
data_a, params_a = hddm.generate.gen_rand_data({'go': level1a,
'nogo': level2a,},
size=trials_per_level,
subjs=n_subjects)
# session 2
level1b = {'v':.6, 'a':1.5, 't':.2,'sv': 0, 'z':.7, 'sz': 0, 'st': 0}
level2b = {'v':-1.5, 'a':1.5, 't':.2,'sv': 0, 'z':.3, 'sz': 0, 'st': 0}
data_b, params_b = hddm.generate.gen_rand_data({'go': level1b,
'nogo': level2b,},
size=trials_per_level,
subjs=n_subjects)
data_a['session'] = Series(np.ones((len(data_a))), index=data_a.index)
data_b['session'] = Series(np.ones((len(data_b)))*2, index=data_a.index)
# we expect v to change between sessions and between go/no-go conditions
data_a['cond_v'] = data_a.condition.str.cat(data_a.session.astype(np.int64).apply(str))
data_b['cond_v'] = data_b.condition.str.cat(data_b.session.astype(np.int64).apply(str))
mydata = data_a.append(data_b, ignore_index=True)
# set noResponse RT to -1, not necessary, but jsut to make things clear
mydata.rt[mydata.response == 0] = -1
mydata.to_csv('mydata.csv')
def z_link_func(x, data=mydata):
stim = (np.asarray(dmatrix('0 + C(s, [[1], [-1]])',
{'s': data.condition.ix[x.index]}))
)
return 1 / (1 + np.exp(-(x * stim)))
# if you assume other parameter changes as well, we need to add other parameters
z_reg = {'model': 'z ~ 1 + C(session)', 'link_func': z_link_func}
v_reg = {'model': 'v ~ 1 + C(cond_v)', 'link_func': lambda x: x}
a_reg = {'model': 'a ~ 1 + C(session)', 'link_func': lambda a: a}
t_reg = {'model': 't ~ 1 + C(session)', 'link_func': lambda t: t}
reg_descr = [z_reg, v_reg, a_reg, t_reg]
m_reg = hddm.HDDMRegressor(mydata, reg_descr, include='z')
m_reg.sample(2000, burn=200)
m_reg.print_stats() # check the outputs in comparision with the true param
"""
Example of one run.
Note that the z values should be passed through the transition function to get its face values.
mean std 2.5q 25q 50q 75q 97.5q mc err
z_Intercept 0.346673 0.0564847 0.239106 0.307751 0.3441 0.386205 0.461575 0.00439742
z_Intercept_std 0.211743 0.0405919 0.124576 0.186462 0.212431 0.240409 0.289452 0.00228978
z_Intercept_subj.0 0.468137 0.076576 0.313579 0.416551 0.469894 0.523116 0.61058 0.00546733
z_Intercept_subj.1 0.2409 0.0505806 0.150262 0.204568 0.238209 0.273871 0.346782 0.00324306
z_Intercept_subj.2 0.271563 0.0573848 0.166577 0.230793 0.269063 0.311191 0.38972 0.00391868
z_Intercept_subj.3 0.310187 0.0620826 0.195229 0.267373 0.306494 0.353033 0.431924 0.00445056
z_Intercept_subj.4 0.44693 0.0736314 0.298593 0.395914 0.449606 0.499134 0.589479 0.00533299
z_C(session)[T.2.0] -0.747035 0.149815 -1.00368 -0.853931 -0.75911 -0.65343 -0.405417 0.0126088
v_Intercept 0.40884 0.119403 0.163848 0.342015 0.404791 0.473467 0.659351 0.00406407
v_Intercept_std 0.2066 0.12635 0.064433 0.126097 0.174526 0.252869 0.55676 0.00568009
v_Intercept_subj.0 0.264072 0.0711253 0.128652 0.215637 0.264595 0.309564 0.403086 0.00345553
v_Intercept_subj.1 0.414372 0.0715001 0.268727 0.369005 0.414065 0.463023 0.55052 0.00309055
v_Intercept_subj.2 0.59832 0.0840216 0.426102 0.541955 0.599277 0.655588 0.768225 0.0038385
v_Intercept_subj.3 0.415052 0.0715428 0.282286 0.363137 0.413303 0.464479 0.558555 0.00344218
v_Intercept_subj.4 0.329264 0.0749482 0.183207 0.280229 0.330535 0.380806 0.475904 0.00348097
v_C(cond_v)[T.go2] 0.385051 0.137392 0.128313 0.291263 0.381375 0.471719 0.679809 0.010247
v_C(cond_v)[T.nogo1] -1.74283 0.0776232 -1.89234 -1.79724 -1.74164 -1.68926 -1.59147 0.00286797
v_C(cond_v)[T.nogo2] -2.90649 0.169356 -3.22299 -3.02155 -2.90799 -2.79034 -2.56435 0.0104097
a_Intercept 2.11514 0.143712 1.85965 2.02601 2.11038 2.18617 2.44977 0.00585757
a_Intercept_std 0.267584 0.159312 0.0991149 0.164557 0.223291 0.319768 0.69504 0.00644995
a_Intercept_subj.0 2.35081 0.0790306 2.19834 2.2965 2.35103 2.40267 2.50895 0.00439321
a_Intercept_subj.1 2.01399 0.0656827 1.89115 1.96844 2.01179 2.05876 2.14611 0.00412558
a_Intercept_subj.2 1.92823 0.0749201 1.79621 1.87649 1.9218 1.97856 2.08789 0.00519325
a_Intercept_subj.3 2.05542 0.0692842 1.91864 2.00723 2.05535 2.10082 2.19216 0.00428725
a_Intercept_subj.4 2.20608 0.0782418 2.05521 2.1526 2.2051 2.25517 2.36135 0.00513966
a_C(session)[T.2.0] -0.683105 0.0684222 -0.820286 -0.727738 -0.687073 -0.634711 -0.552473 0.00522558
t_Intercept 0.348954 0.0578091 0.256088 0.313574 0.342387 0.373827 0.489879 0.00241531
t_Intercept_std 0.115675 0.0716851 0.0479778 0.0729371 0.0956295 0.133578 0.290729 0.0037631
t_Intercept_subj.0 0.338424 0.012289 0.312781 0.330424 0.339155 0.346913 0.360888 0.000548951
t_Intercept_subj.1 0.230491 0.0129194 0.205673 0.220674 0.230349 0.2405 0.254102 0.00106308
t_Intercept_subj.2 0.340683 0.0110646 0.318427 0.333152 0.34061 0.348867 0.361432 0.000819053
t_Intercept_subj.3 0.429096 0.0109785 0.406575 0.42145 0.429563 0.437048 0.449291 0.000752518
t_Intercept_subj.4 0.315421 0.0145723 0.288236 0.30473 0.315293 0.325572 0.343437 0.00114481
t_C(session)[T.2.0] -0.187779 0.0140875 -0.217928 -0.198076 -0.186927 -0.177364 -0.162839 0.00125402
DIC: 2962.471799
deviance: 2938.642003
pD: 23.829796
"""
| 75444e8c620db53280db071904a0a007a8635ab4 | [
"Python"
] | 2 | Python | ccbrain/gongo | e0ecc244cbdb441a9ade0c3d722489ed67d4a9c2 | fd269c0b9b10887f6df74dee7bb7d139869ac189 |
refs/heads/master | <repo_name>eh-project/eh-project<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/HaiGoods.java
package com.ehais.figoarticle.model;
import java.io.Serializable;
import java.math.BigDecimal;
import java.util.Date;
public class HaiGoods implements Serializable {
private Boolean priceWarning;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.goods_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Long goodsId;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.cat_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer catId;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.goods_sn
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String goodsSn;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.goods_name
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String goodsName;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.brand_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer brandId;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.balance_price
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer balancePrice;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.shop_price
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer shopPrice;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.keywords
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String keywords;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.act_desc
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String actDesc;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.goods_thumb
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String goodsThumb;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.goods_img
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String goodsImg;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.original_img
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String originalImg;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.is_on_sale
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Boolean isOnSale;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.add_time
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer addTime;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.sort_order
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Short sortOrder;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.last_update
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer lastUpdate;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.exchangeRate
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private BigDecimal exchangerate;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.profit
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private BigDecimal profit;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.shipping
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private BigDecimal shipping;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.profitType
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer profittype;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.currency
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String currency;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String goodsUrl;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.create_time
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Date createTime;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.updated
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer updated;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.created
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer created;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.deleted
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer deleted;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.website_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer websiteId;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.attr_group
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String attrGroup;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.stock
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer stock;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.fid
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer fid;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.buy_type
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer buyType;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.reserve_price
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Float reservePrice;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.last_price
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Float lastPrice;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.uv
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer uv;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.pv
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer pv;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.salesquantity
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer salesquantity;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.local_thumb
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String localThumb;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.category_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer categoryId;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.spider_source
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String spiderSource;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_goods
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private static final long serialVersionUID = 1L;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.goods_id
*
* @return the value of hai_goods.goods_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Long getGoodsId() {
return goodsId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.goods_id
*
* @param goodsId the value for hai_goods.goods_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setGoodsId(Long goodsId) {
this.goodsId = goodsId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.cat_id
*
* @return the value of hai_goods.cat_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getCatId() {
return catId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.cat_id
*
* @param catId the value for hai_goods.cat_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setCatId(Integer catId) {
this.catId = catId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.goods_sn
*
* @return the value of hai_goods.goods_sn
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getGoodsSn() {
return goodsSn;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.goods_sn
*
* @param goodsSn the value for hai_goods.goods_sn
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setGoodsSn(String goodsSn) {
this.goodsSn = goodsSn;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.goods_name
*
* @return the value of hai_goods.goods_name
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getGoodsName() {
return goodsName;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.goods_name
*
* @param goodsName the value for hai_goods.goods_name
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setGoodsName(String goodsName) {
this.goodsName = goodsName;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.brand_id
*
* @return the value of hai_goods.brand_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getBrandId() {
return brandId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.brand_id
*
* @param brandId the value for hai_goods.brand_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setBrandId(Integer brandId) {
this.brandId = brandId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.balance_price
*
* @return the value of hai_goods.balance_price
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getBalancePrice() {
return balancePrice;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.balance_price
*
* @param balancePrice the value for hai_goods.balance_price
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setBalancePrice(Integer balancePrice) {
this.balancePrice = balancePrice;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.shop_price
*
* @return the value of hai_goods.shop_price
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getShopPrice() {
return shopPrice;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.shop_price
*
* @param shopPrice the value for hai_goods.shop_price
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setShopPrice(Integer shopPrice) {
this.shopPrice = shopPrice;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.keywords
*
* @return the value of hai_goods.keywords
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getKeywords() {
return keywords;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.keywords
*
* @param keywords the value for hai_goods.keywords
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setKeywords(String keywords) {
this.keywords = keywords;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.act_desc
*
* @return the value of hai_goods.act_desc
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getActDesc() {
return actDesc;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.act_desc
*
* @param actDesc the value for hai_goods.act_desc
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setActDesc(String actDesc) {
this.actDesc = actDesc;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.goods_thumb
*
* @return the value of hai_goods.goods_thumb
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getGoodsThumb() {
return goodsThumb;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.goods_thumb
*
* @param goodsThumb the value for hai_goods.goods_thumb
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setGoodsThumb(String goodsThumb) {
this.goodsThumb = goodsThumb;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.goods_img
*
* @return the value of hai_goods.goods_img
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getGoodsImg() {
return goodsImg;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.goods_img
*
* @param goodsImg the value for hai_goods.goods_img
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setGoodsImg(String goodsImg) {
this.goodsImg = goodsImg;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.original_img
*
* @return the value of hai_goods.original_img
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getOriginalImg() {
return originalImg;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.original_img
*
* @param originalImg the value for hai_goods.original_img
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setOriginalImg(String originalImg) {
this.originalImg = originalImg;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.is_on_sale
*
* @return the value of hai_goods.is_on_sale
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Boolean getIsOnSale() {
return isOnSale;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.is_on_sale
*
* @param isOnSale the value for hai_goods.is_on_sale
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setIsOnSale(Boolean isOnSale) {
this.isOnSale = isOnSale;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.add_time
*
* @return the value of hai_goods.add_time
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getAddTime() {
return addTime;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.add_time
*
* @param addTime the value for hai_goods.add_time
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setAddTime(Integer addTime) {
this.addTime = addTime;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.sort_order
*
* @return the value of hai_goods.sort_order
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Short getSortOrder() {
return sortOrder;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.sort_order
*
* @param sortOrder the value for hai_goods.sort_order
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setSortOrder(Short sortOrder) {
this.sortOrder = sortOrder;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.last_update
*
* @return the value of hai_goods.last_update
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getLastUpdate() {
return lastUpdate;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.last_update
*
* @param lastUpdate the value for hai_goods.last_update
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setLastUpdate(Integer lastUpdate) {
this.lastUpdate = lastUpdate;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.exchangeRate
*
* @return the value of hai_goods.exchangeRate
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public BigDecimal getExchangerate() {
return exchangerate;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.exchangeRate
*
* @param exchangerate the value for hai_goods.exchangeRate
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setExchangerate(BigDecimal exchangerate) {
this.exchangerate = exchangerate;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.profit
*
* @return the value of hai_goods.profit
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public BigDecimal getProfit() {
return profit;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.profit
*
* @param profit the value for hai_goods.profit
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setProfit(BigDecimal profit) {
this.profit = profit;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.shipping
*
* @return the value of hai_goods.shipping
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public BigDecimal getShipping() {
return shipping;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.shipping
*
* @param shipping the value for hai_goods.shipping
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setShipping(BigDecimal shipping) {
this.shipping = shipping;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.profitType
*
* @return the value of hai_goods.profitType
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getProfittype() {
return profittype;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.profitType
*
* @param profittype the value for hai_goods.profitType
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setProfittype(Integer profittype) {
this.profittype = profittype;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.currency
*
* @return the value of hai_goods.currency
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getCurrency() {
return currency;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.currency
*
* @param currency the value for hai_goods.currency
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setCurrency(String currency) {
this.currency = currency;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.goods_url
*
* @return the value of hai_goods.goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getGoodsUrl() {
return goodsUrl;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.goods_url
*
* @param goodsUrl the value for hai_goods.goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setGoodsUrl(String goodsUrl) {
this.goodsUrl = goodsUrl;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.create_time
*
* @return the value of hai_goods.create_time
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Date getCreateTime() {
return createTime;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.create_time
*
* @param createTime the value for hai_goods.create_time
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setCreateTime(Date createTime) {
this.createTime = createTime;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.updated
*
* @return the value of hai_goods.updated
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getUpdated() {
return updated;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.updated
*
* @param updated the value for hai_goods.updated
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setUpdated(Integer updated) {
this.updated = updated;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.created
*
* @return the value of hai_goods.created
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getCreated() {
return created;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.created
*
* @param created the value for hai_goods.created
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setCreated(Integer created) {
this.created = created;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.deleted
*
* @return the value of hai_goods.deleted
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getDeleted() {
return deleted;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.deleted
*
* @param deleted the value for hai_goods.deleted
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setDeleted(Integer deleted) {
this.deleted = deleted;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.website_id
*
* @return the value of hai_goods.website_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getWebsiteId() {
return websiteId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.website_id
*
* @param websiteId the value for hai_goods.website_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setWebsiteId(Integer websiteId) {
this.websiteId = websiteId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.attr_group
*
* @return the value of hai_goods.attr_group
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getAttrGroup() {
return attrGroup;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.attr_group
*
* @param attrGroup the value for hai_goods.attr_group
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setAttrGroup(String attrGroup) {
this.attrGroup = attrGroup;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.stock
*
* @return the value of hai_goods.stock
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getStock() {
return stock;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.stock
*
* @param stock the value for hai_goods.stock
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setStock(Integer stock) {
this.stock = stock;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.fid
*
* @return the value of hai_goods.fid
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getFid() {
return fid;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.fid
*
* @param fid the value for hai_goods.fid
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setFid(Integer fid) {
this.fid = fid;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.buy_type
*
* @return the value of hai_goods.buy_type
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getBuyType() {
return buyType;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.buy_type
*
* @param buyType the value for hai_goods.buy_type
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setBuyType(Integer buyType) {
this.buyType = buyType;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.reserve_price
*
* @return the value of hai_goods.reserve_price
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Float getReservePrice() {
return reservePrice;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.reserve_price
*
* @param reservePrice the value for hai_goods.reserve_price
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setReservePrice(Float reservePrice) {
this.reservePrice = reservePrice;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.last_price
*
* @return the value of hai_goods.last_price
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Float getLastPrice() {
return lastPrice;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.last_price
*
* @param lastPrice the value for hai_goods.last_price
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setLastPrice(Float lastPrice) {
this.lastPrice = lastPrice;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.uv
*
* @return the value of hai_goods.uv
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getUv() {
return uv;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.uv
*
* @param uv the value for hai_goods.uv
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setUv(Integer uv) {
this.uv = uv;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.pv
*
* @return the value of hai_goods.pv
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getPv() {
return pv;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.pv
*
* @param pv the value for hai_goods.pv
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setPv(Integer pv) {
this.pv = pv;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.salesquantity
*
* @return the value of hai_goods.salesquantity
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getSalesquantity() {
return salesquantity;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.salesquantity
*
* @param salesquantity the value for hai_goods.salesquantity
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setSalesquantity(Integer salesquantity) {
this.salesquantity = salesquantity;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.local_thumb
*
* @return the value of hai_goods.local_thumb
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getLocalThumb() {
return localThumb;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.local_thumb
*
* @param localThumb the value for hai_goods.local_thumb
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setLocalThumb(String localThumb) {
this.localThumb = localThumb;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.category_id
*
* @return the value of hai_goods.category_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getCategoryId() {
return categoryId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.category_id
*
* @param categoryId the value for hai_goods.category_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setCategoryId(Integer categoryId) {
this.categoryId = categoryId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.spider_source
*
* @return the value of hai_goods.spider_source
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getSpiderSource() {
return spiderSource;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.spider_source
*
* @param spiderSource the value for hai_goods.spider_source
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setSpiderSource(String spiderSource) {
this.spiderSource = spiderSource;
}
public Boolean getPriceWarning() {
return priceWarning;
}
public void setPriceWarning(Boolean priceWarning) {
this.priceWarning = priceWarning;
}
}<file_sep>/school-weixin/src/main/java/com/ehais/schoolweixin/service/userRepairService.java
package com.ehais.schoolweixin.service;
import org.ehais.tools.ReturnObject;
import com.ehais.schoolweixin.model.wxRepair;
public interface userRepairService {
ReturnObject<?> saveRepairList(wxRepair urmodel);
ReturnObject<wxRepair> getRepairList(String wxid);
}<file_sep>/reptileV1/src/main/java/com/ehais/reptile/impl/CommonReptileV2.java
package com.ehais.reptile.impl;
import com.ehais.bean.Brand;
import com.ehais.http.HttpGoods;
public class CommonReptileV2 extends CommonReptile{
protected HttpGoods httpGoods;
public CommonReptileV2() {
// TODO Auto-generated constructor stub
httpGoods = new HttpGoods();
}
public void PrintBrand(Brand brand){
System.out.println("Brand_name = "+brand.getBrand_name());
System.out.println("brand_logo = "+brand.getBrand_logo());
System.out.println("brand_desc = "+brand.getBrand_desc());
System.out.println("site_url = "+brand.getSite_url());
System.out.println("sort_order = "+brand.getSort_order());
System.out.println("is_show = "+brand.getIs_show());
System.out.println("store_id = "+brand.getStore_id());
System.out.println("=========================================");
}
}
<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/service/ScrcService.java
package com.ehais.hrlucene.service;
/**
* @author stephenzhang
*
*/
public interface ScrcService {
public void Loadrc(String positionURL) throws Exception;
}
<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/model/HaiArticleCat.java
package com.ehais.hrlucene.model;
public class HaiArticleCat {
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_article_cat.cat_id
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
private Short cat_id;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_article_cat.cat_name
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
private String cat_name;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_article_cat.cat_type
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
private Boolean cat_type;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_article_cat.keywords
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
private String keywords;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_article_cat.cat_desc
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
private String cat_desc;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_article_cat.sort_order
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
private Byte sort_order;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_article_cat.show_in_nav
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
private Boolean show_in_nav;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_article_cat.parent_id
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
private Short parent_id;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_article_cat.store_id
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
private Integer store_id;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_article_cat.code
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
private String code;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_article_cat.user_id
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
private Long user_id;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_article_cat.images
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
private String images;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_article_cat.cat_id
*
* @return the value of hai_article_cat.cat_id
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public Short getCat_id() {
return cat_id;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_article_cat.cat_id
*
* @param cat_id the value for hai_article_cat.cat_id
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setCat_id(Short cat_id) {
this.cat_id = cat_id;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_article_cat.cat_name
*
* @return the value of hai_article_cat.cat_name
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public String getCat_name() {
return cat_name;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_article_cat.cat_name
*
* @param cat_name the value for hai_article_cat.cat_name
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setCat_name(String cat_name) {
this.cat_name = cat_name;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_article_cat.cat_type
*
* @return the value of hai_article_cat.cat_type
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public Boolean getCat_type() {
return cat_type;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_article_cat.cat_type
*
* @param cat_type the value for hai_article_cat.cat_type
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setCat_type(Boolean cat_type) {
this.cat_type = cat_type;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_article_cat.keywords
*
* @return the value of hai_article_cat.keywords
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public String getKeywords() {
return keywords;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_article_cat.keywords
*
* @param keywords the value for hai_article_cat.keywords
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setKeywords(String keywords) {
this.keywords = keywords;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_article_cat.cat_desc
*
* @return the value of hai_article_cat.cat_desc
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public String getCat_desc() {
return cat_desc;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_article_cat.cat_desc
*
* @param cat_desc the value for hai_article_cat.cat_desc
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setCat_desc(String cat_desc) {
this.cat_desc = cat_desc;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_article_cat.sort_order
*
* @return the value of hai_article_cat.sort_order
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public Byte getSort_order() {
return sort_order;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_article_cat.sort_order
*
* @param sort_order the value for hai_article_cat.sort_order
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setSort_order(Byte sort_order) {
this.sort_order = sort_order;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_article_cat.show_in_nav
*
* @return the value of hai_article_cat.show_in_nav
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public Boolean getShow_in_nav() {
return show_in_nav;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_article_cat.show_in_nav
*
* @param show_in_nav the value for hai_article_cat.show_in_nav
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setShow_in_nav(Boolean show_in_nav) {
this.show_in_nav = show_in_nav;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_article_cat.parent_id
*
* @return the value of hai_article_cat.parent_id
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public Short getParent_id() {
return parent_id;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_article_cat.parent_id
*
* @param parent_id the value for hai_article_cat.parent_id
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setParent_id(Short parent_id) {
this.parent_id = parent_id;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_article_cat.store_id
*
* @return the value of hai_article_cat.store_id
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public Integer getStore_id() {
return store_id;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_article_cat.store_id
*
* @param store_id the value for hai_article_cat.store_id
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setStore_id(Integer store_id) {
this.store_id = store_id;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_article_cat.code
*
* @return the value of hai_article_cat.code
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public String getCode() {
return code;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_article_cat.code
*
* @param code the value for hai_article_cat.code
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setCode(String code) {
this.code = code;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_article_cat.user_id
*
* @return the value of hai_article_cat.user_id
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public Long getUser_id() {
return user_id;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_article_cat.user_id
*
* @param user_id the value for hai_article_cat.user_id
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setUser_id(Long user_id) {
this.user_id = user_id;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_article_cat.images
*
* @return the value of hai_article_cat.images
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public String getImages() {
return images;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_article_cat.images
*
* @param images the value for hai_article_cat.images
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setImages(String images) {
this.images = images;
}
}<file_sep>/eh-common/src/main/java/org/ehais/util/Bean2Utils.java
package org.ehais.util;
import java.beans.BeanInfo;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.beanutils.BeanUtils;
import org.apache.http.NameValuePair;
import org.apache.http.message.BasicNameValuePair;
import org.ehais.annotation.EColumn;
import org.ehais.model.Menu;
/**
* 多种model与map互转对象
* @author tyler
*
*/
public class Bean2Utils {
public static <T> Map<String,Object> printEntity(T obj){
Map<String, Object> map = new HashMap<String, Object>();
try {
BeanInfo beanInfo = Introspector.getBeanInfo(obj.getClass());
PropertyDescriptor[] propertyDescriptors = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor property : propertyDescriptors) {
String key = property.getName();
// 过滤class属性
if (!key.equals("class")) {
// 得到property对应的getter方法
Method getter = property.getReadMethod();
Object value = getter.invoke(obj);
System.out.println(key +" == "+ value);
map.put(key, value);
}
}
} catch (Exception e) {
System.out.println("transBean2Map Error " + e);
}
return map;
}
public static <T> Map<String,Object> toMap(T t){
Map<String,Object> map = new HashMap<String, Object>();
Field[] fields = t.getClass().getDeclaredFields();
for (Field field : fields) {
try {
field.setAccessible(true);
Object obj = field.get(t);
map.put(field.getName(), obj);
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
return map;
}
public static <T> Map<String,String> toMapString(T t){
Map<String,String> map = new HashMap<String, String>();
Field[] fields = t.getClass().getDeclaredFields();
for (Field field : fields) {
try {
field.setAccessible(true);
Object obj = field.get(t);
if(obj!=null)map.put(field.getName(), obj.toString());
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
return map;
}
/**
* 直接返回httpclient post的可用参数
* @param t
* @return
*/
public static <T> List<NameValuePair> toNameValuePairList(T t){
List<NameValuePair> reqEntity = new ArrayList<NameValuePair>();
Field[] fields = t.getClass().getDeclaredFields();
for (Field field : fields) {
try {
field.setAccessible(true);
Object obj = field.get(t);
if(obj!=null)reqEntity.add(new BasicNameValuePair(field.getName(), obj.toString()));
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
return reqEntity;
}
public static <T> Map<String,String> toMapInterfaceString(T t){
Map<String,String> map = new HashMap<String, String>();
Field[] fields = t.getClass().getDeclaredFields();
for (Field field : fields) {
try {
field.setAccessible(true);
Object obj = field.get(t);
EColumn column = field.getAnnotation(EColumn.class);
if(obj!=null && column != null){
map.put(column.name(), obj.toString());
}
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
return map;
}
public static <T> Map<String,Object> beanToMap(T obj){
if(obj == null){
return null;
}
Map<String, Object> map = new HashMap<String, Object>();
try {
BeanInfo beanInfo = Introspector.getBeanInfo(obj.getClass());
PropertyDescriptor[] propertyDescriptors = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor property : propertyDescriptors) {
String key = property.getName();
// 过滤class属性
if (!key.equals("class")) {
// 得到property对应的getter方法
Method getter = property.getReadMethod();
Object value = getter.invoke(obj);
map.put(key, value);
}
}
} catch (Exception e) {
System.out.println("transBean2Map Error " + e);
}
return map;
}
// Map --> Bean 2: 利用org.apache.commons.beanutils 工具类实现 Map --> Bean
public static void transMap2Bean2(Map<String, Object> map, Object obj) {
if (map == null || obj == null) {
return;
}
try {
BeanUtils.populate(obj, map);
} catch (Exception e) {
System.out.println("transMap2Bean2 Error " + e);
}
}
// Map --> Bean 1: 利用Introspector,PropertyDescriptor实现 Map --> Bean
public static void transMap2Bean(Map<String, Object> map, Object obj) {
try {
BeanInfo beanInfo = Introspector.getBeanInfo(obj.getClass());
PropertyDescriptor[] propertyDescriptors = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor property : propertyDescriptors) {
String key = property.getName();
if (map.containsKey(key)) {
Object value = map.get(key);
// 得到property对应的setter方法
Method setter = property.getWriteMethod();
setter.invoke(obj, value);
}
}
} catch (Exception e) {
System.out.println("transMap2Bean Error " + e);
}
return;
}
// Bean --> Map 1: 利用Introspector和PropertyDescriptor 将Bean --> Map
public static Map<String, Object> transBean2Map(Object obj) {
if(obj == null){
return null;
}
Map<String, Object> map = new HashMap<String, Object>();
try {
BeanInfo beanInfo = Introspector.getBeanInfo(obj.getClass());
PropertyDescriptor[] propertyDescriptors = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor property : propertyDescriptors) {
String key = property.getName();
// 过滤class属性
if (!key.equals("class")) {
// 得到property对应的getter方法
Method getter = property.getReadMethod();
Object value = getter.invoke(obj);
map.put(key, value);
}
}
} catch (Exception e) {
System.out.println("transBean2Map Error " + e);
}
return map;
}
public static Object mapToBean(Map<String, Object> map, Class cls) throws Exception
{
Object object = cls.newInstance();
for (String key : map.keySet()){
Field temFiels = cls.getDeclaredField(key);
temFiels.setAccessible(true);
temFiels.set(object, map.get(key));
}
return object;
}
public static void main(String[] args) {
Menu menu = new Menu();
menu.setId("xdf");
menu.setTitle("wq lsdf");
// Map<String,Object> map = Bean2Utils.beanToMap(menu);
Map<String,Object> map = Bean2Utils.toMap(menu);
for (Map.Entry<String,Object> m : map.entrySet()) {
System.out.println(m.getKey()+"=="+m.getValue());
}
//
// Map<String, Object> mp = new HashMap<String, Object>();
// mp.put("name", "Mike");
// mp.put("age", 25);
// mp.put("mN", "male");
//
// // 将map转换为bean
// transMap2Bean2(mp, person);
//
// System.out.println("--- transMap2Bean Map Info: ");
// for (Map.Entry<String, Object> entry : mp.entrySet()) {
// System.out.println(entry.getKey() + ": " + entry.getValue());
// }
//
// System.out.println("--- Bean Info: ");
// System.out.println("name: " + person.getName());
// System.out.println("age: " + person.getAge());
// System.out.println("mN: " + person.getmN());
//
// // 将javaBean 转换为map
// Map<String, Object> map = transBean2Map(person);
//
// System.out.println("--- transBean2Map Map Info: ");
// for (Map.Entry<String, Object> entry : map.entrySet()) {
// System.out.println(entry.getKey() + ": " + entry.getValue());
// }
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/mapper/BrandMapper.java
package com.ehais.figoarticle.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
import com.ehais.figoarticle.model.Brand;
import com.ehais.figoarticle.model.BrandExample;
public interface BrandMapper {
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
@Select("select count(*) from brand where #{field} = #{value} ")
int unique(@Param("field") String field, @Param("value") String value);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
@Select("select count(*) from brand where #{field} = #{value} and store_id = #{store_id}")
int uniqueStore(@Param("field") String field, @Param("value") String value, @Param("store_id") Integer store_id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
int countByExample(BrandExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
int deleteByExample(BrandExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
int deleteByPrimaryKey(Integer id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
int insert(Brand record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
int insertSelective(Brand record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
List<Brand> selectByExample(BrandExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
Brand selectByPrimaryKey(Integer id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
int updateByExampleSelective(@Param("record") Brand record, @Param("example") BrandExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
int updateByExample(@Param("record") Brand record, @Param("example") BrandExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
int updateByPrimaryKeySelective(Brand record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table brand
*
* @mbggenerated Sun Mar 19 20:19:27 CST 2017
*/
int updateByPrimaryKey(Brand record);
}<file_sep>/reptileV1/src/main/java/com/ehais/reptile/impl/ShopBopImpl.java
package com.ehais.reptile.impl;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.ehais.tools.ReturnObject;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.junit.Test;
import com.ehais.bean.Goods;
import com.ehais.reptile.Reptile;
import net.sf.json.JSONArray;
public class ShopBopImpl extends CommonReptile implements Reptile{
public ShopBopImpl() {
// TODO Auto-generated constructor stub
host = "http://cn.shopbop.com";
}
@Override
@Test
public void list(){
String url = "";
url = "http://cn.shopbop.com/shoes-booties/br/v=1/2534374302112431.htm";
url = "http://cn.shopbop.com/bags/br/v=1/2534374302024667.htm";
try {
this.product_list(url);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
public ReturnObject<Object> product_list(String url) throws Exception{
System.out.println("开始抓取网址,请等候...");
System.out.println(url);
System.out.println();
ReturnObject<Object> rm = new ReturnObject<Object>();
List<Object> list = new ArrayList<Object>();
String contents = httpClient.methodGet(url);
// System.out.println("contents:"+contents);
Document doc = Jsoup.parse(contents);
Element content = doc.getElementById("product-container");
if(content == null)return null;
Elements ul_li = content.getElementsByTag("li");
if(ul_li == null)return null;
String href = "";
String title = "";
for(Element li : ul_li){
Elements li_a = li.getElementsByTag("a");
href = host + li_a.first().attr("href");
title = li.getElementsByAttributeValue("class", "title").text();
System.out.println("产品title:"+title);
System.out.println("产品href:"+href);
Map<String ,String> map = new HashMap<String, String>();
map.put("href", href);
map.put("title", title);
list.add(map);
// Elements td = tds.getElementsByTag("td");
// if(td == null || td.equals(""))return null;
// if(td.size() == 4){
// for(Element product : td){
// if(product == null)return null;
//// this.ProductElement(product);
// href = product.getElementsByAttributeValue("class", "productDetailLink").attr("href");
// title = product.getElementsByAttributeValue("class", "productTitle").html();
// System.out.println("产品title:"+title);
// System.out.println("产品href:"+href);
// Map<String ,String> map = new HashMap<String, String>();
// map.put("href", host + href);
// map.put("title", title);
// list.add(map);
// }
// }
}
// Element nextPageLink = doc.getElementsByAttributeValue("class", "nextPageLink").first();
//
// if(nextPageLink.toString() != null || !nextPageLink.toString().equals("")){
// url = this.get_next_link(nextPageLink.attr("onclick").toString());
// if(url!=null && !url.equals("")){
// rm.setNextLink(host+url);
// }
// }
rm.setRows(list);
return rm;
}
@Test
public void info(){
String url = "http://cn.shopbop.com/rosa-solid-cashmere-tunic-dress/vp/v=1/1570614449.htm?folderID=2534374302029428&fm=other-shopbysize&colorId=18618";
url = "http://cn.shopbop.com/medium-tote-bag-lesportsac/vp/v=1/1558062517.htm?folderID=2534374302024667&fm=other&colorId=12867";
try {
this.product_info(url);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
public ReturnObject<Object> product_info(String url) throws Exception{
System.out.println("获取商品详情链接,请等候...");
System.out.println(url);
System.out.println();
ReturnObject<Object> rm = new ReturnObject<Object>();
Goods goods = new Goods();
goods.setGoodsUrl(url);
String contents = httpClient.methodGet(url);
Document doc = Jsoup.parse(contents);
Element product_information = doc.getElementById("product-information");
String brand = product_information.getElementsByTag("a").first().getElementsByTag("h1").html();
goods.setBrandName(brand);
String name = product_information.getElementsByTag("p").first().html();
goods.setGoodsName(name);
String price = product_information.getElementById("productPrices").getElementsByTag("meta").first().attr("content");
goods.setPrice(price.substring(1, price.length()).trim().replaceAll(",", ""));
//样本
Element swatches = doc.getElementById("swatches");
List<String> map_swatches = this.get_attribute_swatches_list(swatches);
JSONArray colorObj = JSONArray.fromObject(map_swatches);
System.out.println(colorObj.toString());
goods.setColorJson(colorObj.toString());
//尺寸
Element sizeContainer = doc.getElementById("sizes");
List<String> map_sizeContainer = this.get_attribute_sizeContainer_list(sizeContainer);
JSONArray sizeObj = JSONArray.fromObject(map_sizeContainer);
System.out.println(sizeObj.toString());
goods.setSizeJson(sizeObj.toString());
String productImage = doc.getElementById("productImage").attr("src");
goods.setImage(productImage);
Element detailsAccordion = doc.getElementById("detailsAccordion");
String content = detailsAccordion.getElementsByTag("div").first().getElementsByAttributeValue("class", "content").first().html();
// System.out.println("tontent:"+content);
goods.setGoods_desc(content);
//相册
Element thumbnailList = doc.getElementById("thumbnailList");
List<String> goodsGallery = this.get_goods_gallery_list(thumbnailList);
goods.setGoodsImage(goodsGallery);
//分类
Element CategoryEl = doc.getElementsByAttributeValue("class", "breadcrumbs").first();
String category = this.get_Category(CategoryEl);
goods.setCategoryName(category);
category = null;
CategoryEl = null;
//商品编号
Element goods_sn = doc.getElementById("offerSku").getElementsByTag("span").first();
goods.setGoodsSN(goods_sn.text());
System.out.println("goods_sn:"+goods.getGoodsSN());
rm.setModel(goods);
this.PrintlnGoods(goods);
return rm;
}
@Override
//样本
public Map<String,String> get_attribute_swatches(Element swatches){
Map<String,String> map_attribute_swatches = new HashMap<String,String>();
Elements img = swatches.getElementsByTag("img");
String src = "";
String title = "";
for (Element element : img) {
src = element.attr("src");
title = element.attr("title");
map_attribute_swatches.put(title, src);
}
return map_attribute_swatches;
}
@Override
//样本
public List<String> get_attribute_swatches_list(Element swatches){
List<String> map_attribute_swatches = new ArrayList<String>();
Elements img = swatches.getElementsByTag("img");
String src = "";
String title = "";
for (Element element : img) {
src = element.attr("src");
title = element.attr("title");
map_attribute_swatches.add(title);
}
return map_attribute_swatches;
}
@Override
//尺寸
public Map<String,String> get_attribute_sizeContainer(Element sizeContainer){
Map<String,String> map_attribute_size = new HashMap<String,String>();
Elements span = sizeContainer.getElementsByTag("span");
String data_selectedsize = "";
String id = "";
for (Element element : span) {
data_selectedsize = element.attr("data-selectedsize");
id = element.attr("id");
map_attribute_size.put(id, data_selectedsize);
}
return map_attribute_size;
}
@Override
//尺寸
public List<String> get_attribute_sizeContainer_list(Element sizeContainer){
List<String> map_attribute_size = new ArrayList<String>();
Elements span = sizeContainer.getElementsByTag("span");
String data_selectedsize = "";
String id = "";
for (Element element : span) {
data_selectedsize = element.attr("data-selectedsize");
id = element.attr("id");
map_attribute_size.add( data_selectedsize);
}
return map_attribute_size;
}
@Override
//获取下一页列表
public String get_next_link(String href){
Pattern pattern = Pattern.compile("'(.*?)'");
Matcher matcher = pattern.matcher(href);
String url = "";
while(matcher.find()){
url = matcher.group(1);
}
System.out.println("下一页:"+url);
return url;
}
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
// ShopBopImp shop = new ShopBopImp();
// shop.whats_new_list();
System.out.println("ShopBop....");
}
@Override
public List<String> get_goods_gallery_list(Element goodsGallery) {
// TODO Auto-generated method stub
Elements liGG = goodsGallery.getElementsByTag("li").attr("class", "thumbnailListItem");
List<String> gg = new ArrayList<String>();
String img = "";
for (Element element : liGG) {
img = element.getElementsByTag("img").attr("src").toString();
if(img.indexOf("_37x65")>0){
img = img.replaceAll("_37x65", "");
System.out.println("相册:"+img);
gg.add(img);
}
}
return gg;
}
@Override
public String get_Category(Element category) {
// TODO Auto-generated method stub
if(category == null)return "";
Elements span = category.getElementsByTag("li");
String cat_name = "";
for(Element s : span){
cat_name += s.text()+",";
}
if(cat_name.length()>0){
cat_name = cat_name.substring(0,cat_name.length() - 1);
}
System.out.println("分类:"+cat_name);
return cat_name;
}
@Override
public List<Map<String, String>> get_goods_gallery_list_Map(
Element goodsGallery) {
// TODO Auto-generated method stub
return null;
}
}
<file_sep>/reptileV1/src/main/java/com/ehais/reptile/ReptileRunnable.java
package com.ehais.reptile;
import java.util.List;
import java.util.Map;
import org.eclipse.swt.widgets.Text;
import org.ehais.tools.ReturnObject;
import com.ehais.bean.Goods;
import com.ehais.http.HttpGoods;
import com.ehais.reptile.impl.LanecrawfordImpl;
import com.ehais.reptile.impl.MytheresaImpl;
import com.ehais.reptile.impl.Net_A_PorterImpl;
import com.ehais.reptile.impl.ShopBopImpl;
import com.ehais.reptile.impl.StyleBopImpl;
public class ReptileRunnable implements Runnable {
private ShopBopImpl shopbop;
private MytheresaImpl mytheresa;
private Net_A_PorterImpl net_a_porter;
private LanecrawfordImpl lanecrawford;
private StyleBopImpl stylebop;
private String url;
private Integer type;
private ReturnObject<Object> rm;
private Text text_result;
private Goods goods;
private HttpGoods httpGoods;
private String result;
private String category_name;
private String currency;
private String exchangeRate;
private String shipping;
private String profit;
private int profitType;
public ReptileRunnable(Text text_result) {
// TODO Auto-generated constructor stub
shopbop = new ShopBopImpl();
mytheresa = new MytheresaImpl();
net_a_porter = new Net_A_PorterImpl();
lanecrawford = new LanecrawfordImpl();
stylebop = new StyleBopImpl();
url = "";
type = 0;
rm = new ReturnObject<Object>();
httpGoods = new HttpGoods();
this.text_result = text_result;
}
public void setUrl(String url){
this.url = url;
}
public void setType(Integer type){
this.type = type;
}
public void setCategory_name(String category_name) {
this.category_name = category_name;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public void setExchangeRate(String exchangeRate) {
this.exchangeRate = exchangeRate;
}
public void setShipping(String shipping) {
this.shipping = shipping;
}
public void setProfit(String profit) {
this.profit = profit;
}
public void setProfitType(int profitType) {
this.profitType = profitType;
}
@Override
public void run() {
// TODO Auto-generated method stub
try{
this.ProductList(url);
if(rm!=null){
if(type == 0){
List<Object> list = rm.getRows();
for (Object object : list) {
Map<String,String> map = (Map<String, String>) object;
if(Thread.currentThread().getName().equals("shopbop")){
ReturnObject<Object> rm_product = shopbop.product_info(shopbop.getHost() + map.get("href"));
this.ProductPost(rm_product);
}else if(Thread.currentThread().getName().equals("mytheresa")){
ReturnObject<Object> rm_product = mytheresa.product_info(map.get("href"));
this.ProductPost(rm_product);
}else if(Thread.currentThread().getName().equals("net-a-porter")){
ReturnObject<Object> rm_product = net_a_porter.product_info(map.get("href"));
this.ProductPost(rm_product);
}else if(Thread.currentThread().getName().equals("lanecrawford")){
ReturnObject<Object> rm_product = lanecrawford.product_info(map.get("href"));
this.ProductPost(rm_product);
}else if(Thread.currentThread().getName().equals("stylebop")){
ReturnObject<Object> rm_product = stylebop.product_info(map.get("href"));
this.ProductPost(rm_product);
}
}
if(Thread.currentThread().getName().equals("shopbop")){
}else{
}
}else{
this.ProductPost(rm);
}
}
}catch(Exception e){
e.printStackTrace();
//补充上传信息
}
}
public void ProductList(String url) throws Exception{
if(Thread.currentThread().getName().equals("shopbop")){
if(type == 0){
rm = shopbop.product_list(url);
}else{
rm = shopbop.product_info(url);
}
}else if(Thread.currentThread().getName().equals("mytheresa")){
if(type == 0){
rm = mytheresa.product_list(url);
}else{
rm = mytheresa.product_info(url);
}
}else if(Thread.currentThread().getName().equals("net-a-porter")){
if(type == 0){
rm = net_a_porter.product_list(url);
}else{
rm = net_a_porter.product_info(url);
}
}else if(Thread.currentThread().getName().equals("lanecrawford")){
if(type == 0){
rm = lanecrawford.product_list(url);
}else{
rm = lanecrawford.product_info(url);
}
}else if(Thread.currentThread().getName().equals("stylebop")){
if(type == 0){
rm = stylebop.product_list(url);
}else{
rm = stylebop.product_info(url);
}
}
if(rm!=null){
if(type == 0){
List<Object> list = rm.getRows();
for (Object object : list) {
Map<String,String> map = (Map<String, String>) object;
if(Thread.currentThread().getName().equals("shopbop")){
ReturnObject<Object> rm_product = shopbop.product_info(map.get("href"));
this.ProductPost(rm_product);
}else if(Thread.currentThread().getName().equals("mytheresa")){
ReturnObject<Object> rm_product = mytheresa.product_info(map.get("href"));
this.ProductPost(rm_product);
}else if(Thread.currentThread().getName().equals("net-a-porter")){
ReturnObject<Object> rm_product = net_a_porter.product_info(map.get("href"));
this.ProductPost(rm_product);
}else if(Thread.currentThread().getName().equals("lanecrawford")){
ReturnObject<Object> rm_product = lanecrawford.product_info(map.get("href"));
this.ProductPost(rm_product);
}else if(Thread.currentThread().getName().equals("stylebop")){
ReturnObject<Object> rm_product = stylebop.product_info(map.get("href"));
this.ProductPost(rm_product);
}
}
// if(rm.getNextLink()!=null && !rm.getNextLink().equals("")){
// this.ProductList(rm.getNextLink());
// }
}
}
}
public void ProductPost(ReturnObject<Object> rm_product){
goods = (Goods) rm_product.getModel();
if(goods == null) return ;
if(goods.getCategoryName() == null || goods.getCategoryName().equals(""))goods.setCategoryName("");
// goods.setCategoryName(this.category_name);
goods.setCurrency(this.currency);
goods.setExchangeRate(this.exchangeRate);
goods.setProfit(this.profit);
goods.setProfitType(this.profitType+"");
goods.setShipping(this.shipping);
result = httpGoods.postGoods(goods);
// text_result.setText(text_result.getText()+ "提交结果:"+result+"\r\n");
text_result.getDisplay().asyncExec(new Runnable(){
public void run() {
// TODO Auto-generated method stub
text_result.setText("名称:"+goods.getGoodsName()+"\r\n" +
"价格:"+goods.getPrice()+"\r\n" +
"品牌:"+goods.getBrandName()+"\r\n" +
"颜色:"+goods.getColorJson()+"\r\n" +
"尺寸:"+goods.getSizeJson()+"\r\n" +
"提交结果:"+result+"\r\n\r\n"+
text_result.getText());
}
});
}
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
}
}
<file_sep>/eh-common/src/main/java/org/ehais/service/impl/verifyCodeComfirmImpl.java
package org.ehais.service.impl;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.ehais.service.verifyCodeComfirm;
import org.ehais.tools.ReturnObject;
import org.springframework.stereotype.Service;
import org.springframework.ui.ModelMap;
@Service("verifyCodeComfirm")
public class verifyCodeComfirmImpl implements verifyCodeComfirm {
/* (non-Javadoc)
* @see org.ehais.service.impl.verifyCodeComfirm#confirmCode(javax.servlet.http.HttpServletRequest, org.springframework.ui.ModelMap)
*/
public ReturnObject<?> confirmCode(HttpServletRequest request){
HttpSession session=request.getSession();
String verifyCode=(String)session.getAttribute("validateCode");
String userCode=request.getParameter("verify");
System.out.println("userCode-->"+userCode);
System.out.println("verifyCode-->"+verifyCode);
ReturnObject<?> rm = new ReturnObject<>();
if(!(verifyCode.equalsIgnoreCase(userCode))){
rm.setCode(-3);
//rm.setMsg("验证码不正确");
}
else{
rm.setCode(1);
}
return rm;
}
}
<file_sep>/eh-common/src/main/java/org/ehais/util/ECommon.java
package org.ehais.util;
import java.math.BigDecimal;
import java.text.DecimalFormat;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.commons.lang3.RandomStringUtils;
public class ECommon {
/**
* 判断bigDecimal数据是否为空,如果传入参数是null,则返回"0"
*
* @param bd
* 需要判断的bigDecimal数据
* @return 返回参数对应的bigDecimal数据
*/
public static BigDecimal checkBigDecimal(BigDecimal bd) {
if (bd == null) {
bd = new BigDecimal(0);
}
return bd;
}
/**
* 判断bigDecimal数据是否为空,如果传入参数是null,则返回"0"
*
* @param bd
* 需要判断的bigDecimal数据
* @return 返回参数对应的bigDecimal数据
*/
public static Double checkDouble(Double bd) {
if (bd == null) {
bd = new Double(0);
}
return bd;
}
/**
* 取给定的字符串长度和传入长度比较,相等返回TRUE,否则返回FALSE.
*
* @param str1
* 待比较字符串
* @param length
* 串长
* @return
*/
public static Boolean checkStringLength(String str1, int length) {
if (null == str1 || length < 0) {
return Boolean.FALSE;
}
return Boolean.valueOf(str1.length() == length);
}
/**
* 将给定的字符串长度转换成4位长度字符串,不足4位前边补零。
*
* @param strLen
* @return
*/
public static String getLength(int strLen) {
String str = String.valueOf(strLen);
for (int i = str.length(); i < 4; i++) {
str = "0" + str;
}
return str;
}
/**
* 判断bigDecimal数据是否为空,如果传入参数是null,则返回"0"
*
* @param bd
* 需要判断的bigDecimal数据
* @return 返回参数对应的double数据
*/
public static double convertBDtoD(BigDecimal bd) {
if (bd == null) {
bd = new BigDecimal(0);
}
return bd.doubleValue();
}
/**
* 将给定的字符串数组转换成特定格式的字符串
*
* @param info
* 字符串数组
* @return
*/
public static String getSocketOutputInfo(String[] info) {
StringBuffer sb = new StringBuffer(1024);
// 将数组中的数据存入字符串缓冲区
for (int i = 0; i < info.length; i++) {
sb.append("|");
sb.append(info[i]);
}
// 计算纯字符串数据长度
// by zhangwd 20070105
// int length = sb.toString().trim().length();
int length = sb.toString().length();
// 将长度补到生成的串前
sb.insert(0, getLength(length + 4));
return sb.toString();
}
/**
* 将字符串以某一个特定字符串分离开
*
* @param tmpMsg
* 待分离字符串
* @param separater
* 分隔符
* @return
*/
public static String[] getStringArrayBySomeString(String tmpMsg,
String separater) {
List msgFieldList = new ArrayList();
while (tmpMsg != null && !tmpMsg.equals("")) {
int firstD = tmpMsg.indexOf(separater);
if (firstD == -1) {
msgFieldList.add(tmpMsg);
tmpMsg = null;
} else if (firstD == 0) {
msgFieldList.add("");
tmpMsg = tmpMsg.substring(firstD + 1);
} else {
msgFieldList.add(tmpMsg.substring(0, firstD));
tmpMsg = tmpMsg.substring(firstD + 1);
}
}
String[] fields = new String[msgFieldList.size()];
int ii = 0;
for (Iterator it = msgFieldList.iterator(); it.hasNext();) {
fields[ii++] = (String) it.next();
}
return fields;
}
/**
* 固定字符串填充
*
* @param str
* 源串
* @param length
* 固定长度
* @param c
* 填充位置
* @param fillChar
* 填充字符
* @return
*/
public static String getCertainLengthString(String str, int length,
Character c, Character fillChar) {
char temStr[] = new char[length];
for (int i = 0; i < temStr.length; i++)
temStr[i] = fillChar.charValue();
if (str == null || str.trim().length() == 0)
return String.valueOf(temStr);
if (str.getBytes().length > length)
return str.substring(0, length);
char inputStr[] = new char[length - str.getBytes().length];
for (int j = 0; j < length - str.getBytes().length; j++)
inputStr[j] = fillChar.charValue();
if (c.charValue() == 'L')
return str.concat(String.valueOf(inputStr));
if (c.charValue() == 'R')
return String.valueOf(inputStr).concat(str);
else
return null;
}
/**
* 获取当前系统时间,精确到纳秒
*
* @return
*/
public static String getCurrentTime() {
Date dnow = new Date();
SimpleDateFormat formatter = new SimpleDateFormat("HHmmssSSSSSS");
return formatter.format(dnow);
}
public static String getStrFromByteArray(byte[] src, int srcPos, int length) {
byte[] tempArray = new byte[length];
System.arraycopy(src, srcPos, tempArray, 0, length);
return new String(tempArray);
}
/**
* 将金额转换成不带小数点的字符串,如30.56经过转换后变为3056
*
* @param bd
* @return
*/
public static String convertMoneytoString(Double bd) {
DecimalFormat df = new DecimalFormat("######.00");
String result = String.valueOf(df.format(100D * checkDouble(bd)
.doubleValue()));
if (result.indexOf(".") != -1)
return result.substring(0, result.indexOf("."));
else
return result;
}
/**
* 将金额转换成小数点两位小数的字符串,如30.5经过转换后变为30.50
* 0.1 经过转换后变为 0.10
* @param bd
* @return
*/
public static String convertMoneytoString2(Double bd) {
DecimalFormat df = new DecimalFormat("0.00");
String result = String.valueOf(df.format(checkDouble(bd)
.doubleValue()));
return result;
}
/**
* 判断字符串是否数字
* @param str
* @return
*/
public static boolean isNumeric(String str){
Pattern pattern = Pattern.compile("[0-9]*");
Matcher isNum = pattern.matcher(str);
if( !isNum.matches() ){
return false;
}
return true;
}
/**
* 日期正则表达式
* @param dateStr
* @return
*/
public static boolean isDate(String dateStr){
Pattern p = Pattern.compile("(\\d{1,4}[-|\\/|年|\\.]\\d{1,2}[-|\\/|月|\\.]\\d{1,2}([日|号])?(\\s)*(\\d{1,2}([点|时])?((:)?\\d{1,2}(分)?((:)?\\d{1,2}(秒)?)?)?)?(\\s)*(PM|AM)?)", Pattern.CASE_INSENSITIVE|Pattern.MULTILINE);
Matcher matcher = p.matcher(dateStr);
if( !matcher.matches() ){
return false;
}
return true;
}
/**
* 产生len位的随机数
* @param len
* @return
*/
public static String nonceStr(int len){
return RandomStringUtils.random(len,"abcdefthijklmnopqrstuvwxyz1234567890");
}
public static String nonceStrUpper(int len){
return RandomStringUtils.random(len,"abcdefthijklmnopqrstuvwxyz1234567890ABCDEFGHIJKLMNOPQRSTUVWXYZ");
}
public static String nonceInt(int len){
return RandomStringUtils.random(len,"1234567890");
}
}
<file_sep>/reptileV1/pom.xml
<?xml version="1.0"?>
<project xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd" xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>com.ehais</groupId>
<artifactId>ehproject</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<groupId>com.ehais</groupId>
<artifactId>reptileV1</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>reptileV1</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
<!-- <dependency>
<groupId>httpunit</groupId>
<artifactId>httpunit</artifactId>
<version>1.7</version>
</dependency> -->
<dependency>
<groupId>net.sourceforge.htmlunit</groupId>
<artifactId>htmlunit-core-js</artifactId>
<version>2.15</version>
</dependency>
<dependency>
<groupId>net.sourceforge.htmlunit</groupId>
<artifactId>htmlunit</artifactId>
<version>2.15</version>
</dependency>
<dependency>
<groupId>xml-apis</groupId>
<artifactId>xml-apis</artifactId>
<version>1.4.01</version>
</dependency>
<dependency>
<groupId>rhino</groupId>
<artifactId>js</artifactId>
<version>1.7R2</version>
</dependency>
<dependency>
<groupId>javax.activation</groupId>
<artifactId>activation</artifactId>
<version>1.1</version>
</dependency>
<dependency>
<groupId>net.sourceforge.nekohtml</groupId>
<artifactId>nekohtml</artifactId>
<version>1.9.21</version>
</dependency>
<dependency>
<groupId>xerces</groupId>
<artifactId>xercesImpl</artifactId>
<version>2.11.0</version>
</dependency>
<dependency>
<groupId>xerces</groupId>
<artifactId>xmlParserAPIs</artifactId>
<version>2.6.2</version>
</dependency>
<dependency>
<groupId>org.jsoup</groupId>
<artifactId>jsoup</artifactId>
<version>1.7.2</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.3.5</version>
</dependency>
<dependency>
<groupId>net.sf.json-lib</groupId>
<artifactId>json-lib</artifactId>
<version>2.4</version>
<classifier>jdk15</classifier>
</dependency>
<!--
<dependency>
<groupId>ehais</groupId>
<artifactId>haisoftware</artifactId>
<version>1.0</version>
</dependency>
-->
<dependency>
<groupId>com.ehais</groupId>
<artifactId>eh-common</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>swt</groupId>
<artifactId>swt-win32</artifactId>
<version>3.0m8</version>
</dependency>
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
<version>1.9</version>
</dependency>
<dependency>
<groupId>commons-collections</groupId>
<artifactId>commons-collections</artifactId>
<version>3.2.1</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.4</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
<dependency>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
<version>1.1.3</version>
</dependency>
<dependency>
<groupId>net.sourceforge.cssparser</groupId>
<artifactId>cssparser</artifactId>
<version>0.9.14</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.3.2</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpcore</artifactId>
<version>4.3.2</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpmime</artifactId>
<version>4.3.3</version>
</dependency>
<dependency>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-http</artifactId>
<version>8.1.15.v20140411</version>
</dependency>
<dependency>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-io</artifactId>
<version>8.1.15.v20140411</version>
</dependency>
<dependency>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-util</artifactId>
<version>8.1.15.v20140411</version>
</dependency>
<dependency>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-websocket</artifactId>
<version>8.1.15.v20140411</version>
</dependency>
<dependency>
<groupId>org.w3c.css</groupId>
<artifactId>sac</artifactId>
<version>1.3</version>
</dependency>
<dependency>
<groupId>xalan</groupId>
<artifactId>serializer</artifactId>
<version>2.7.1</version>
</dependency>
<dependency>
<groupId>dom4j</groupId>
<artifactId>dom4j</artifactId>
<version>1.6.1</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.1</version>
<configuration>
<source>1.7</source>
<target>1.7</target>
<encoding>UTF-8</encoding>
</configuration>
</plugin>
</plugins>
</build>
</project>
<file_sep>/eh-weixin/src/main/java/org/ehais/weixin/EConstants.java
package org.ehais.weixin;
import org.ehais.util.ResourceUtil;
public class EConstants {
// public static String weixin_appid = ResourceUtil.getProValue("weixin_appid");
// public static String weixin_appsecret = ResourceUtil.getProValue("weixin_appsecret");
// public static String weixin_mch_id = ResourceUtil.getProValue("weixin_mch_id");
// public static String weixin_mch_secret = ResourceUtil.getProValue("weixin_mch_secret");
public static String wxdev_token = "<PASSWORD>";//ResourceUtil.getProValue("wxdev_token");
// 获取access_token的接口地址(GET) 限200(次/天)
public final static String access_token_url = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=APPID&secret=APPSECRET";
// 菜单创建(POST) 限100(次/天)
public static String menu_create_url = "https://api.weixin.qq.com/cgi-bin/menu/create?access_token=ACCESS_TOKEN";
// 组成可以获取授权的链接
public static String authorize = "https://open.weixin.qq.com/connect/oauth2/authorize?appid=APPID&redirect_uri=REDIRECT_URI&response_type=code&scope=SCOPE&state=STATE#wechat_redirect";
// 获取opendid的接口地址
public static String get_opendid_url = "https://api.weixin.qq.com/sns/oauth2/access_token?grant_type=authorization_code";
// 获取用户信息,并判断是否关注此微信号
public static String get_user_info = "https://api.weixin.qq.com/cgi-bin/user/info?access_token=ACCESS_TOKEN&openid=OPENID&lang=zh_CN";
// 获取ip
public static String get_call_back_ip = "https://api.weixin.qq.com/cgi-bin/getcallbackip?access_token=ACCESS_TOKEN";
// 获取getJsApiTicket
public static String get_jsapi_url = "https://api.weixin.qq.com/cgi-bin/ticket/getticket?type=jsapi&access_token=ACCESS_TOKEN&type=jsapi";
// 统一下单
public static String unifiedorder = "https://api.mch.weixin.qq.com/pay/unifiedorder";
// 退款申请
public static String refund_url = "https://api.mch.weixin.qq.com/secapi/pay/refund";
// 退款查询
public static String refund_query_url = "https://api.mch.weixin.qq.com/pay/refundquery";
// 发送红包
public static String send_red_pack = "https://api.mch.weixin.qq.com/mmpaymkttransfers/sendredpack";
// 上传媒体
public static String upload_media = "http://file.api.weixin.qq.com/cgi-bin/media/upload?access_token=ACCESS_TOKEN&type=TYPE";
// 下载媒体
public static String download_media = "http://file.api.weixin.qq.com/cgi-bin/media/get?access_token=ACCESS_TOKEN&media_id=MEDIA_ID";
//上传图片
public static String upload_img = "https://api.weixin.qq.com/cgi-bin/media/uploadimg?access_token=ACCESS_TOKEN";
//上传图文消息
public static String upload_news = "https://api.weixin.qq.com/cgi-bin/media/uploadnews?access_token=ACCESS_TOKEN";
//
//创建微信分组
public static String groups_create = "https://api.weixin.qq.com/cgi-bin/groups/create?access_token=ACCESS_TOKEN";
//修改微信分组
public static String groups_update = "https://api.weixin.qq.com/cgi-bin/groups/update?access_token=ACCESS_TOKEN";
//删除微信分组
public static String groups_delete = "https://api.weixin.qq.com/cgi-bin/groups/delete?access_token=ACCESS_TOKEN";
//根据分组进行群发消息
public static String mass_sendall = "https://api.weixin.qq.com/cgi-bin/message/mass/sendall?access_token=ACCESS_TOKEN";
//创建二维码
public static String qrcode = "https://api.weixin.qq.com/cgi-bin/qrcode/create?access_token=TOKENPOST";
//创建短码
public static String shorturl = "https://api.weixin.qq.com/cgi-bin/shorturl?access_token=ACCESS_TOKEN";
//使用该接口,商户可获取账号下的类目与号段等信息
public static String merchantinfo = "https://api.weixin.qq.com/scan/merchantinfo/get?access_token=TOKEN";
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/DemoController.java
package com.ehais.figoarticle.controller;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.lang3.StringEscapeUtils;
import org.ehais.controller.CommonController;
import org.ehais.util.Bean2Utils;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.mapper.HaiBrandMapper;
import com.ehais.figoarticle.mapper.HaiCategoryMapper;
import com.ehais.figoarticle.mapper.HaiGoodsMapper;
import com.ehais.figoarticle.mapper.HaiGoodsUrlMapper;
import com.ehais.figoarticle.model.HaiCategory;
import com.ehais.figoarticle.model.HaiCategoryExample;
import com.ehais.figoarticle.model.HaiGoodsAttr;
import com.ehais.figoarticle.model.HaiGoodsEntity;
import com.ehais.figoarticle.model.HaiGoodsGallery;
import com.ehais.figoarticle.model.HaiGoodsUrl;
import com.ehais.figoarticle.model.HaiGoodsUrlExample;
import com.ehais.figoarticle.model.HaiGoodsWithBLOBs;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
@Controller
@RequestMapping("/demo")
public class DemoController extends FigoCommonController {
private static String url = "https://www.ifchic.com/";
@ResponseBody
@RequestMapping("/brand")
public String brand(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
return "";
}
@ResponseBody
@RequestMapping("/category")
public String category(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
this.category(request, url);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private void category(HttpServletRequest request,String categoryUrl){
String result = "";
try {
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), categoryUrl);
// result = PythonUtil.python("D:/workspace_jee/figoarticle/src/main/webapp/getAjaxWeb.py", categoryUrl);
// result = FSO.ReadFileName("E:/temp/IFCHIC.htm");
Document doc = Jsoup.parse(result);
List<HaiCategory> list = new ArrayList<HaiCategory>();
// TODO
System.out.println("==========================================");
System.out.println("==========================================");
JSONArray arr = JSONArray.fromObject(list);
System.out.println(arr.toString());
// System.out.println(element.html());
System.out.println("==========================================");
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/category";
// String api = "http://localhost:8087/api/category";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
}
@ResponseBody
@RequestMapping("/url")
public String url(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
c.andCategoryUrlLike(url+"%");
List<HaiCategory> listCategory = haiCategoryMapper.selectByExample(example);
for (HaiCategory haiCategory : listCategory) {
System.out.println(haiCategory.getCategoryUrl());
this.goodsUrl(request, haiCategory.getCategoryUrl(), haiCategory.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsUrl(HttpServletRequest request,String goodsurl,Integer catId){
System.out.println(goodsurl);
String result = "";
try{
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
// result = PythonUtil.python("D:/workspace_jee/figoarticle/src/main/webapp/getAjaxWeb.py", categoryUrl);
// result = FSO.ReadFileName("E:/temp/IFCHIC.htm");
Document doc = Jsoup.parse(result);
List<String> list = new ArrayList<String>();
// TODO
JSONArray arr = JSONArray.fromObject(list);
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("catId", catId.toString());
paramsMap.put("json", arr.toString());
// String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/url";
String api = "http://localhost:8087/api/url";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
//获取下一页
/**
Element page_chooser = page.getElementById("page-chooser");
Element next = page_chooser.getElementsByClass("next").first();
if(next != null){
String href_a = next.attr("href");
this.goodsUrl(request, href_a, catId);
}
**/
}catch(Exception e){
e.printStackTrace();
}
return result;
}
@ResponseBody
@RequestMapping("/goodsAll")
public String goodsAll(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiGoodsUrlExample example = new HaiGoodsUrlExample();
HaiGoodsUrlExample.Criteria c = example.createCriteria();
c.andGoodsUrlLike(url+"%");
List<HaiGoodsUrl> listGoodsUrl = haiGoodsUrlMapper.selectByExample(example);
for (HaiGoodsUrl haiGoodsUrl : listGoodsUrl) {
goodsModel(request,haiGoodsUrl.getGoodsUrl(),haiGoodsUrl.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
public String goodsModel(HttpServletRequest request , String goodsurl ,Integer catId){
System.out.println(goodsurl);
String result = "";
HaiGoodsEntity entity = new HaiGoodsEntity();
HaiGoodsWithBLOBs goods = new HaiGoodsWithBLOBs();
List<HaiGoodsGallery> goodsGalleryList = new ArrayList<HaiGoodsGallery>();
List<HaiGoodsAttr> goodsAttrList = new ArrayList<HaiGoodsAttr>();
HaiGoodsAttr goodsAttr = new HaiGoodsAttr();
goods.setGoodsUrl(goodsurl);
goods.setCatId(catId);
try{
Bean2Utils.printEntity(goods);
entity.setGoods(goods);
entity.setGoodsAttrList(goodsAttrList);
entity.setGoodsGalleryList(goodsGalleryList);
JSONObject jsonObject = JSONObject.fromObject(entity);
System.out.println(jsonObject.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", jsonObject.toString());
// String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/goods";
// String api = "http://localhost:8087/api/goods";
// String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
public static void main(String[] args) throws Exception {
String goodsurl = "https://www.net-a-porter.com/cn/zh/d/Shop/Lingerie/All?cm_sp=topnav-_-clothing-_-lingerie";
DemoController ac = new DemoController();
// ac.goodsModel(url,1);
ac.goodsUrl(null, goodsurl, 1);
}
}
<file_sep>/src/main/resources/config/application-dev.properties
jndiName=java:comp/env/jndi/ehproject<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/GebnegozionlineController.java
package com.ehais.figoarticle.controller;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.logging.LogFactory;
import org.ehais.controller.CommonController;
import org.ehais.util.EHtmlUnit;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.FSO;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.model.HaiCategory;
import com.ehais.figoarticle.model.HaiCategoryExample;
import com.ehais.figoarticle.model.HaiGoodsAttr;
import com.ehais.figoarticle.model.HaiGoodsEntity;
import com.ehais.figoarticle.model.HaiGoodsGallery;
import com.ehais.figoarticle.model.HaiGoodsUrl;
import com.ehais.figoarticle.model.HaiGoodsUrlExample;
import com.ehais.figoarticle.model.HaiGoodsWithBLOBs;
import com.gargoylesoftware.htmlunit.BrowserVersion;
import com.gargoylesoftware.htmlunit.NicelyResynchronizingAjaxController;
import com.gargoylesoftware.htmlunit.WebClient;
import com.gargoylesoftware.htmlunit.html.HtmlAnchor;
import com.gargoylesoftware.htmlunit.html.HtmlPage;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
@Controller
@RequestMapping("/gebnegozionline")
public class GebnegozionlineController extends FigoCommonController {
private String url = "https://www.gebnegozionline.com";
private int websiteId = 7;
@ResponseBody
@RequestMapping("/category")
public String category(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
// this.category(request, "https://www.gebnegozionline.com/en/pages/index/woman-6/");
// this.category(request, "https://www.gebnegozionline.com/en/pages/index/man-5/");
this.category(request, url);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private void category(HttpServletRequest request,String categoryUrl){
String result = "";
try {
// TODO
List<HaiCategory> list = new ArrayList<HaiCategory>();
result = this.webRequest(request, categoryUrl, "webclient");
Document doc = Jsoup.parse(result);
Element mainMenu = doc.getElementsByClass("topbar__main-sections__items").first();
Elements lis = mainMenu.getElementsByTag("li");
for(Element li : lis) {
Element la = li.getElementsByTag("a").first();
String name = la.text();
String href = la.attr("href");
if(href.indexOf("http") < 0)
href = url + href;
System.out.println(href);
HaiCategory cat = new HaiCategory();
cat.setCatName(name);
cat.setCategoryUrl(href);
cat.setIsShow(true);
cat.setWebsiteId(websiteId);
//子分类
List<HaiCategory> catList = new ArrayList<HaiCategory>();
//Document doc1 = Jsoup.connect(href).get();
// // 模拟浏览器打开一个目标网址
// final HtmlPage page1 = webClient.getPage(href);
// // 该方法在getPage()方法之后调用才能生效
// webClient.waitForBackgroundJavaScript(1000*3);
// webClient.setJavaScriptTimeout(0);
// result = page1.asXml();
result = this.webRequest(request, href, "webclient");
// result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), href);
Document doc1 = Jsoup.parse(result);
//二级菜单
Element customercareMenuContainer = doc1.getElementById("customercareMenuContainer");
Element menuUl = customercareMenuContainer.getElementsByTag("ul").first();
Elements childLis = menuUl.select(">li");
//System.out.println(childLis);
//System.out.println(childLis.size());
childLis.remove(0);
for(Element childLi : childLis) {
Element childa = childLi.select(">a").first();
String childName = childa.text();
System.out.println(childName);
String childHref = childa.attr("href");
if(childHref.indexOf("http") < 0)
childHref = url + childHref;
HaiCategory cat2 = new HaiCategory();
cat2.setCatName(childName);
cat2.setCategoryUrl(childHref);
cat2.setIsShow(true);
cat2.setParentId(cat.getParentId());
cat2.setWebsiteId(websiteId);
//三级菜单
List<HaiCategory> catList1 = new ArrayList<HaiCategory>();
if(!childName.equals("New Arrivals") && !childName.equals("New arrivals")) {
Element secondUl = childLi.getElementsByClass("expandable-menu__second-level").first();
Elements secondLis = secondUl.getElementsByTag("li");
for(Element secondLi : secondLis) {
Element secondA = secondLi.getElementsByTag("a").first();
String secondName = secondA.text();
String secondHref = secondA.attr("href");
if(secondHref.indexOf("http") < 0)
secondHref = url + secondHref;
System.out.println(secondHref);
HaiCategory cat3= new HaiCategory();
cat3.setCatName(secondName);
cat3.setCategoryUrl(secondHref);
cat3.setIsShow(true);
cat3.setParentId(cat2.getParentId());
cat3.setWebsiteId(websiteId);
catList1.add(cat3);
}
}
cat2.setChildren(catList1);
catList.add(cat2);
}
cat.setChildren(catList);
list.add(cat);
}
System.out.println("==========================================");
System.out.println("==========================================");
JSONArray arr = JSONArray.fromObject(list);
System.out.println(arr.toString());
System.out.println("==========================================");
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", arr.toString());
// String api = "http://localhost:8087/api/category";
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/category";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
}
@ResponseBody
@RequestMapping("/url")
public String url(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
c.andCategoryUrlLike(url+"%");
List<HaiCategory> listCategory = haiCategoryMapper.selectByExample(example);
for (HaiCategory haiCategory : listCategory) {
System.out.println(haiCategory.getCategoryUrl());
this.goodsUrl(request, haiCategory.getCategoryUrl(), haiCategory.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsUrl(HttpServletRequest request,String goodsurl,Integer catId){
System.out.println(goodsurl);
String result = "";
try{
List<String> list = new ArrayList<String>();
// TODO
Document doc = Jsoup.connect(goodsurl).timeout(10000).get();
// result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
// this.fso_write(goodsurl, result);
// Document doc = Jsoup.parse(result);
if(doc == null)
return "";
Element productsGrid = doc.getElementsByClass("products-grid").first();
if(productsGrid == null)
return "";
Elements as = productsGrid.getElementsByClass("product-item__link");
for(Element a : as) {
String aLink = a.attr("href");
if(aLink.indexOf("http") < 0)
aLink = aLink + url;
System.out.println(aLink);
list.add(aLink);
}
JSONArray arr = JSONArray.fromObject(list);
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("catId", catId.toString());
paramsMap.put("json", arr.toString());
// String api = "http://localhost:8087/api/url";
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/url";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
//获取下一页
System.out.println("test1");
Element pagination = doc.getElementsByClass("pagination").first();
if(pagination == null)
return "";
Elements uls = pagination.getElementsByTag("ul");
if(uls == null)
return "";
Elements lis = uls.get(1).getElementsByTag("li");
if(lis == null)
return "";
System.out.println("test2");
System.out.println(lis);
for(Element nextA : lis) {
if(nextA.text().equals("Next") && nextA != null) {
String na = nextA.attr("href");
if(na.indexOf("http") < 0)
na = na + url;
this.goodsUrl(request, na, catId);
}
}
}catch(Exception e){
e.printStackTrace();
}
return result;
}
@ResponseBody
@RequestMapping("/goodsAll")
public String goodsAll(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiGoodsUrlExample example = new HaiGoodsUrlExample();
HaiGoodsUrlExample.Criteria c = example.createCriteria();
c.andGoodsUrlLike(url+"%");
c.andFinishEqualTo(false);
List<HaiGoodsUrl> listGoodsUrl = haiGoodsUrlMapper.selectByExample(example);
for (HaiGoodsUrl haiGoodsUrl : listGoodsUrl) {
goodsModel(request,haiGoodsUrl.getGoodsUrl(),haiGoodsUrl.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
public String goodsModel(HttpServletRequest request , String goodsurl ,Integer catId){
System.out.println(goodsurl);
HaiGoodsEntity entity = new HaiGoodsEntity();
HaiGoodsWithBLOBs goods = new HaiGoodsWithBLOBs();
List<HaiGoodsGallery> goodsGalleryList = new ArrayList<HaiGoodsGallery>();
List<HaiGoodsAttr> goodsAttrList = new ArrayList<HaiGoodsAttr>();
goods.setGoodsUrl(goodsurl);
goods.setCatId(catId);
goods.setWebsiteId(websiteId);
String result = "";
try{
Document doc = Jsoup.connect(goodsurl).timeout(10000).get();
// result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
// this.fso_write(goodsurl, result);
// Document doc = Jsoup.parse(result);
//System.out.println(doc);
if(doc == null)
return "";
Element product = doc.select(".main-area__main-column.main-area__right-column.product-page__details").first();
if(product == null)
return "";
Element brand = product.select(".product-page__details__brand.bold.text-uppercase.f110").first();
System.out.println(brand);
Element nameA = brand.getElementsByTag("a").first();
//设置商品名字
if(nameA == null)
return "";
System.out.println(nameA.text());
goods.setGoodsName(nameA.text());
//设置价格
Integer pri = 0;
Element priceBox = product.select(".price-box__item.price-box--regular").first();
System.out.println(priceBox);
Element currency = priceBox.getElementsByClass("currency").first();
String curr = currency.text();
System.out.println(curr);
goods.setCurrency(curr);
Element span = currency.nextElementSibling();
String price = span.text();
int index = price.indexOf('.');
if(index < 0) {
int index1 = price.indexOf(',');
pri = Integer.parseInt(price.substring(0, index1));
} else {
int index1 = price.indexOf(',');
int thousand = Integer.parseInt(price.substring(0,index)) * 1000 ;
int hundred = Integer.parseInt(price.substring(index + 1, index1));
pri = thousand + hundred;
}
System.out.println(pri);
goods.setShopPrice(pri);
//设置颜色
Element colors = doc.getElementsByClass("product-detail__color-select").first();
Elements options = colors.getElementsByTag("option");
for(Element option : options) {
if(option.hasAttr("disabled"))
continue;
String color = option.text();
HaiGoodsAttr haiGoodsColor = new HaiGoodsAttr();
haiGoodsColor.setAttrType("color");
haiGoodsColor.setAttrValue(color);
haiGoodsColor.setAttrPrice(pri.toString());
goodsAttrList.add(haiGoodsColor);
}
//设置尺寸
Element sizes = doc.getElementsByClass("product-detail__size-select").first();
Elements sizeOptions = sizes.getElementsByTag("option");
for(Element sizeOption : sizeOptions) {
if(sizeOption.hasAttr("disabled"))
continue;
String size = sizeOption.text();
HaiGoodsAttr haiGoodsSize = new HaiGoodsAttr();
haiGoodsSize.setAttrType("size");
haiGoodsSize.setAttrValue(size);
haiGoodsSize.setAttrPrice(pri.toString());
goodsAttrList.add(haiGoodsSize);
}
//设置图片
Element picture = doc.select(".product-image__items.swiper-wrapper").first();
Elements imgs = picture.getElementsByTag("img");
for(Element img : imgs) {
HaiGoodsGallery gallery = new HaiGoodsGallery();
gallery.setThumbUrl(img.attr("src"));
gallery.setImgUrl(img.attr("src"));
gallery.setImgOriginal(img.attr("src"));
goodsGalleryList.add(gallery);
}
if(goodsGalleryList.size() > 0) {
HaiGoodsGallery gallery = goodsGalleryList.get(0);
goods.setGoodsThumb(gallery.getThumbUrl());
goods.setGoodsImg(gallery.getImgUrl());
goods.setOriginalImg(gallery.getImgOriginal());
}
entity.setGoods(goods);
entity.setGoodsAttrList(goodsAttrList);
entity.setGoodsGalleryList(goodsGalleryList);
JSONObject jsonObject = JSONObject.fromObject(entity);
System.out.println(jsonObject.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", jsonObject.toString());
// String api = "http://localhost:8087/api/goodsAttr";
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/goodsAttr";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
public static void main(String[] args){
}
@ResponseBody
@RequestMapping("/brand")
public String brand(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
String url = "https://www.gebnegozionline.com/en/pages/index/man-15/?show=all";
// url = "http://www.toutiao.com/search_content/?format=json&keyword=apple";
// url = "http://www.ehais.com";
url = "https://www.baidu.com/s?ie=utf-8&f=8&rsv_bp=1&rsv_idx=2&tn=baiduhome_pg&wd=java%20httpunit%20https&oq=java%25E8%25B0%2583%25E7%2594%25A8python&rsv_pq=c24f5f6a0002b171&rsv_t=495cVYGnbFnYuGWze7LMWRF2G%2BXAzm4DIgdpf5f%2BxhWBumQ24DfPLD9UazU0gsf1kSOD&rqlang=cn&rsv_enter=1&inputT=6016&rsv_sug3=45&rsv_sug1=22&rsv_sug7=100&rsv_sug2=0&rsv_sug4=6017";
String result = "";
try{
// result = EHtmlUnit.httpUnitRequest(url);
// result = EHtmlUnit.httpUnitAjaxRequest(url);
// result = EHttpClientUtil.methodGet(url);
// System.out.println(result);
// Document doc = Jsoup.parse(result);
// Elements category = doc.getElementsByClass("expandable-menu__first-level").first().getElementsByClass("expandable-menu__first-level__item");
// for (Element element : category) {
// String a = element.getElementsByTag("a").first().text();
// System.out.println(a);
// }
System.out.println("start");
Process pr = Runtime.getRuntime().exec("python D:\\xampp\\thinkcmfx\\getAjaxWeb.py "+url);
BufferedReader in = new BufferedReader(new InputStreamReader( pr.getInputStream()));
String line;
while ((line = in.readLine()) != null) {
System.out.println(line);
result+=line;
}
in.close();
pr.waitFor();
System.out.println("end");
return result;
}catch(Exception e){
e.printStackTrace();
return this.errorJSON(e);
}
}
@ResponseBody
@RequestMapping("/youku")
public String youku(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try{
String url = "http://v.youku.com/v_show/id_XNDc2MDkzMTIw.html";
String xurl = "http://v.youku.com/v_vpofficiallistv5/id_119023280_showid_271942_page_2?__rt=1&__ro=listitem_page2";
// String a = "<a page=\"2\">178-101</a>";
// String url="http://www.baidu.com";
// 模拟一个浏览器
final WebClient webClient = new WebClient(BrowserVersion.FIREFOX_45);
LogFactory.getFactory().setAttribute("org.apache.commons.logging.Log","org.apache.commons.logging.impl.NoOpLog");
java.util.logging.Logger.getLogger("net.sourceforge.htmlunit").setLevel(java.util.logging.Level.OFF);
webClient.getOptions().setThrowExceptionOnScriptError(false);
webClient.getOptions().setThrowExceptionOnFailingStatusCode(false);
// final WebClient webClient=new
// WebClient(BrowserVersion.FIREFOX_10,"http://myproxyserver",8000);
// //使用代理
// final WebClient webClient2=new
// WebClient(BrowserVersion.INTERNET_EXPLORER_10);
// 设置webClient的相关参数
webClient.getOptions().setJavaScriptEnabled(true);
webClient.getOptions().setActiveXNative(false);
webClient.getOptions().setCssEnabled(false);
webClient.getOptions().setThrowExceptionOnScriptError(false);
webClient.waitForBackgroundJavaScript(600*1000);
webClient.setAjaxController(new NicelyResynchronizingAjaxController());
webClient.getOptions().setJavaScriptEnabled(true);
/*
webClient.setJavaScriptTimeout(3600*1000);
webClient.getOptions().setRedirectEnabled(true);
webClient.getOptions().setThrowExceptionOnScriptError(true);
webClient.getOptions().setThrowExceptionOnFailingStatusCode(true);
webClient.getOptions().setTimeout(3600*1000);
webClient.waitForBackgroundJavaScript(600*1000);
*/
// webClient.waitForBackgroundJavaScript(600*1000);
webClient.setAjaxController(new NicelyResynchronizingAjaxController());
// 模拟浏览器打开一个目标网址
final HtmlPage page = webClient.getPage(url);
// 该方法在getPage()方法之后调用才能生效
webClient.waitForBackgroundJavaScript(1000*3);
webClient.setJavaScriptTimeout(0);
String html = page.asXml();
System.out.println(html);
// Thread.sleep(1000 *3L);
// String js = "javascript:checkShowFollow('271942','2');";
// ScriptResult sr = page.executeJavaScript(js);
// HtmlPage newPage = (HtmlPage) sr.getNewPage();
// System.out.println("new page.asText=" + newPage.asText());
// System.out.println("page.asText=" + page.asText());
// System.out.println("page.getUrl=" + page.getUrl());
List links = (List) page.getByXPath ("//*[@id=\"groups_tab\"]/div[1]/ul/li[1]/a");
if(null!=links){
System.out.println(links.size());
HtmlAnchor link = (HtmlAnchor) links.get(0);
System.out.println(link.asXml());
HtmlPage p = link.click();
webClient.waitForBackgroundJavaScript(1000*3L);
// webClient.waitForBackgroundJavaScriptStartingBefore(1000L);
// Thread.sleep(3000L);
System.out.println(p.asText());
}
}catch(Exception e){
e.printStackTrace();
}
return "aa";
}
//单商品的地址请求
@ResponseBody
@RequestMapping("/getGoodsUrl")
public String getGoodsUrl(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "catId", required = true) Integer catId,
@RequestParam(value = "goodsurl", required = true) String goodsurl
){
try{
return goodsModel(request,goodsurl,catId);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
}<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/FarfetchController.java
package com.ehais.figoarticle.controller;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.logging.LogFactory;
import org.ehais.util.Bean2Utils;
import org.ehais.util.EHtmlUnit;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.FSO;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.model.HaiCategory;
import com.ehais.figoarticle.model.HaiCategoryExample;
import com.ehais.figoarticle.model.HaiGoodsAttr;
import com.ehais.figoarticle.model.HaiGoodsEntity;
import com.ehais.figoarticle.model.HaiGoodsGallery;
import com.ehais.figoarticle.model.HaiGoodsUrl;
import com.ehais.figoarticle.model.HaiGoodsUrlExample;
import com.ehais.figoarticle.model.HaiGoodsWithBLOBs;
import com.gargoylesoftware.htmlunit.BrowserVersion;
import com.gargoylesoftware.htmlunit.NicelyResynchronizingAjaxController;
import com.gargoylesoftware.htmlunit.WebClient;
import com.gargoylesoftware.htmlunit.html.HtmlPage;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
//完成
@Controller
@RequestMapping("/farfetch")
public class FarfetchController extends FigoCommonController{
private static String url = "https://www.farfetch.com";
private int websiteId = 5;
@ResponseBody
@RequestMapping("/brand")
public String brand(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
return "";
}
@ResponseBody
@RequestMapping("/category")
public String category(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
String categoryUrl = "https://www.farfetch.com/hk/shopping/women/items.aspx";
this.category(request, categoryUrl);
categoryUrl = "https://www.farfetch.com/hk/shopping/men/items.aspx";
this.category(request, categoryUrl);
categoryUrl = "https://www.farfetch.com/hk/shopping/kids/items.aspx";
this.category(request, categoryUrl);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private void category(HttpServletRequest request,String categoryUrl){
String result = "";
try {
result = EHtmlUnit.getAjaxPage(categoryUrl);
// result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), categoryUrl);
// this.fso_write(categoryUrl, result);
// FSO.WriteTextFile("E:/temp/Farfetchx.html", result);
//result = PythonUtil.python("D:/workspace_jee/figoarticle/src/main/webapp/getAjaxWeb.py", categoryUrl);
// result = FSO.ReadFileName("E:/temp/Farfetch.html");
//System.out.println(result);
this.category_content(request, result);
}catch(Exception e){
e.printStackTrace();
}
}
public void category_content(HttpServletRequest request,String result){
String cateUrl = "";
try {
// Document doc = Jsoup.connect(categoryUrl).get();
Document doc = Jsoup.parse(result);
List<HaiCategory> list = new ArrayList<HaiCategory>();
Element header_nav = doc.getElementById("header-nav");
System.out.println("header_nav.size:"+header_nav.html());
Elements nav = header_nav.getElementsByTag("nav");
System.out.println("nav.size:"+nav.size());
for (Element element : nav) {
Elements ff_primary_nav = element.getElementsByClass("ff-primary-nav");
System.out.println("ff_primary_nav.size:"+ff_primary_nav.size());
for (Element element0 : ff_primary_nav) {
// Elements ff_has_children = element0.getElementsByClass("ff-primary-nav");
Elements ff_has_children = element0.getElementsByTag("li");
System.out.println("ff_has_children.size:"+ff_has_children.size());
for (Element element2 : ff_has_children) {
HaiCategory cate = new HaiCategory();
Element a0 = element2.select(">a").first();
if(a0==null)continue;
cate.setCatName(a0.text());
cate.setCategoryUrl(a0.attr("href").indexOf("http") >= 0 ? a0.attr("href") : (url+a0.attr("href")));
cate.setWebsiteId(websiteId);
//得到每一个分类的数据
if(cate.getCategoryUrl() != null) {
cateUrl = cate.getCategoryUrl();
}
Bean2Utils.printEntity(cate);
list.add(cate);
System.out.println("////////////////////////");
}
}
}
System.out.println("==========================================");
System.out.println("==========================================");
JSONArray arr = JSONArray.fromObject(list);
System.out.println(arr.toString());
// System.out.println(element.html());
System.out.println("==========================================");
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/category";
// String api = "http://localhost:8087/api/category";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
}
//得到每个分类的
private void getArticlesToSaveFromCategory(String url) {
try {
Document doc = Jsoup.connect(url).get();
Element section = doc.getElementsByTag("section").get(1);
//System.out.println("section " + section);
Elements articles = section.getElementsByTag("article");
for(Element article : articles) {
//System.out.println("article" + article);
// System.out.println("===========");
Elements as = article.getElementsByTag("a");
if(as != null)
for(Element a : as) {
System.out.println("a====== " + a);
}
}
}catch(IOException e) {
e.printStackTrace();
}
}
@ResponseBody
@RequestMapping("/url")
public String url(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
c.andCategoryUrlLike(url+"%");
List<HaiCategory> listCategory = haiCategoryMapper.selectByExample(example);
for (HaiCategory haiCategory : listCategory) {
System.out.println(haiCategory.getCategoryUrl());
this.goodsUrl(request, haiCategory.getCategoryUrl(), haiCategory.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsUrl(HttpServletRequest request,String goodsurl,Integer catId){
System.out.println(goodsurl);
String result = "";
try{
result = EHtmlUnit.getAjaxPage(goodsurl);
// result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
// this.fso_write(goodsurl, result);
// result = PythonUtil.python("C:/Users/cjc/Desktop/eh-project/FigoShop/getAjaxWeb.py", goodsurl);
//System.out.println("result = " + result);
// result = FSO.ReadFileName("E:/temp/farfetch.html");
// Document doc = Jsoup.connect(goodsurl).get();
Document doc = Jsoup.parse(result);
// System.out.println("doc = " + doc);
Elements section = doc.getElementsByTag("section");
List<String> list = new ArrayList<String>();
for (Element element : section) {
if(element.attr("class") != null && !element.attr("class").equals("") && !element.attr("class").equals("footer")){
Elements articles = element.getElementsByTag("article");
// System.out.println("articles" + articles);
for(Element article : articles) {
// System.out.println("article" + article);
String ahref = url + article.getElementsByTag("a").first().attr("href");
System.out.println(ahref);
list.add(ahref);
}
//System.out.println(element.attr("class"));
}
}
JSONArray arr = JSONArray.fromObject(list);
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("catId", catId.toString());
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/url";
// String api = "http://localhost:8087/api/url";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
return result;
}
@ResponseBody
@RequestMapping("/goodsAll")
public String goodsAll(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiGoodsUrlExample example = new HaiGoodsUrlExample();
HaiGoodsUrlExample.Criteria c = example.createCriteria();
c.andGoodsUrlLike(url+"%");
c.andFinishEqualTo(false);
List<HaiGoodsUrl> listGoodsUrl = haiGoodsUrlMapper.selectByExample(example);
for (HaiGoodsUrl haiGoodsUrl : listGoodsUrl) {
goodsModel(request,haiGoodsUrl.getGoodsUrl(),haiGoodsUrl.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
public String goodsModel(HttpServletRequest request , String goodsurl ,Integer catId){
System.out.println(goodsurl);
String result = "";
HaiGoodsEntity entity = new HaiGoodsEntity();
HaiGoodsWithBLOBs goods = new HaiGoodsWithBLOBs();
List<HaiGoodsGallery> goodsGalleryList = new ArrayList<HaiGoodsGallery>();
List<HaiGoodsAttr> goodsAttrList = new ArrayList<HaiGoodsAttr>();
HaiGoodsAttr goodsAttr = new HaiGoodsAttr();
goods.setGoodsUrl(goodsurl);
goods.setCatId(catId);
goods.setWebsiteId(websiteId);
try{
result = EHtmlUnit.getAjaxPage(goodsurl);
// result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
// this.fso_write(goodsurl, result);
// result = PythonUtil.python("D:/workspace_jee/GIT/eh-project/FigoShop/getAjaxWeb.py", goodsurl);
// result = FSO.ReadFileName("E:/temp/farfetch.html");
// FSO.WriteTextFile("E:/temp/farfetch2.html", result);
Document doc = Jsoup.parse(result);
String goodsName = "";
String prices = "";
Elements meta = doc.getElementsByTag("meta");
for (Element e : meta) {
if(e.attr("itemProp").equals("name")) {
goodsName = e.attr("content");
System.out.println("商品名称:"+e.attr("content"));
}else if(e.attr("itemProp").equals("price")) {
prices = e.attr("content");
System.out.println("商品价格:"+prices);
}
if(e.attr("property").equals("og:image")) {
HaiGoodsGallery gallery = new HaiGoodsGallery();
gallery.setImgOriginal(e.attr("content"));
gallery.setImgUrl(e.attr("content"));
gallery.setThumbUrl(e.attr("content"));
goodsGalleryList.add(gallery);
}
}
goods.setGoodsName(goodsName);
Float price = Float.parseFloat(prices) * 100;
goods.setShopPrice(price.intValue());
goods.setCurrency("¥");
String goodsDesc = "";
Elements div = doc.getElementsByTag("div");
for (Element e : div) {
if(e.attr("data-tstid").equals("productDetails")) {
goodsDesc = e.html();
}
}
goods.setGoodsDesc(goodsDesc);
// Elements imgLi = doc.select("li.sliderProduct-slide");
// for (Element element : imgLi) {
// Element img = element.getElementsByTag("img").first();
// if(img.attr("data-medium") != null){
// String goodsImg = img.attr("data-medium");
// HaiGoodsGallery gallery = new HaiGoodsGallery();
// gallery.setImgOriginal(goodsImg);
// gallery.setImgUrl(goodsImg);
// gallery.setThumbUrl(goodsImg);
// goodsGalleryList.add(gallery);
// }
// }
if(goodsGalleryList.size() > 0){
HaiGoodsGallery gallery = goodsGalleryList.get(0);
goods.setGoodsThumb(gallery.getThumbUrl());
goods.setGoodsImg(gallery.getImgUrl());
goods.setOriginalImg(gallery.getImgOriginal());
}
Bean2Utils.printEntity(goods);
entity.setGoods(goods);
entity.setGoodsAttrList(goodsAttrList);
entity.setGoodsGalleryList(goodsGalleryList);
JSONObject jsonObject = JSONObject.fromObject(entity);
System.out.println(jsonObject.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", jsonObject.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/goodsAttr";
// String api = "http://localhost:8087/api/goods";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
//单商品的地址请求
@ResponseBody
@RequestMapping("/getGoodsUrl")
public String getGoodsUrl(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "catId", required = true) Integer catId,
@RequestParam(value = "goodsurl", required = true) String goodsurl
){
try{
return goodsModel(request,goodsurl,catId);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
public static void main(String[] args) throws Exception {
String goodsurl = "https://www.farfetch.com/hk/shopping/women/items.aspx";
FarfetchController ac = new FarfetchController();
goodsurl = "https://www.farfetch.com/hk/shopping/kids/msgm-kids--t--item-12114745.aspx?storeid=10238&from=listing&rnkdmnly=1";
ac.goodsModel(null,goodsurl,1);
// goodsurl = "https://www.farfetch.com/hk/shopping/women/clothing-1/items.aspx?ffref=hd_snav";
// ac.goodsUrl(null, goodsurl, 1);
// ac.getArticlesToSaveFromCategory("https://www.farfetch.com/hk/sets/women/new-in-this-week-eu-women.aspx?ffref=hd_mnav");
// ac.category(null, goodsurl);
}
}
<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/service/impl/GgxhService.java
package com.ehais.hrlucene.service.impl;
/**
* @author stephen
*
*/
public interface GgxhService {
public void mainScrape() throws Exception;
}<file_sep>/eh-common/src/main/java/org/ehais/util/SignUtil.java
package org.ehais.util;
import java.io.UnsupportedEncodingException;
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
public class SignUtil {
public static String getSign(Map<String,Object> map,String secret) throws UnsupportedEncodingException{
ArrayList<String> list = new ArrayList<String>();
for(Map.Entry<String,Object> entry:map.entrySet()){
if(entry.getValue()!=""){
list.add(entry.getKey() + "=" + entry.getValue() + "&");
}
}
int size = list.size();
String [] arrayToSort = list.toArray(new String[size]);
Arrays.sort(arrayToSort, String.CASE_INSENSITIVE_ORDER);
StringBuilder sb = new StringBuilder();
for(int i = 0; i < size; i ++) {
sb.append(arrayToSort[i]);
}
String result = sb.toString();
result += "key=" + secret;
// System.out.println(result);
System.out.println("Sign Before MD5:" + result);
result = EncryptUtils.md5(result).toUpperCase();
System.out.println("Sign Result:" + result);
return result;
}
/**
* 自定义的排序map后进行签名
* @param map
* @param secret
* @return
* @throws UnsupportedEncodingException
*/
public static String getSignWS(Map<String,String> map,String secret) throws UnsupportedEncodingException{
ArrayList<String> list = new ArrayList<String>();
for(Map.Entry<String,String> entry:map.entrySet()){
if(entry.getValue()!=""){
list.add(entry.getKey());
}
}
int size = list.size();
String [] arrayToSort = list.toArray(new String[size]);
Arrays.sort(arrayToSort, String.CASE_INSENSITIVE_ORDER);
StringBuilder sb = new StringBuilder();
for(int i = 0; i < size; i ++) {
sb.append(map.get(arrayToSort[i])!=null?map.get(arrayToSort[i]):"");
}
sb.append(secret);
String result = sb.toString();
// result += "key=" + Configure.getKey();
// System.out.println(result);
System.out.println("Sign Before MD5:" + result);
result = EncryptUtils.md5(result).toUpperCase();
System.out.println("Sign Result:" + result);
return result;
}
}
<file_sep>/reptileV1/src/main/java/com/ehais/reptile/impl/MytheresaImpl.java
package com.ehais.reptile.impl;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.ehais.tools.ReturnObject;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.junit.Test;
import com.ehais.bean.Goods;
import com.ehais.reptile.Reptile;
import net.sf.json.JSONArray;
public class MytheresaImpl extends CommonReptile implements Reptile{
public MytheresaImpl() {
// TODO Auto-generated constructor stub
host = "http://www.mytheresa.com";
// /en-de/new-arrivals/what-s-new-this-week-1.html#
}
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
}
@Override
@Test
public void list() {
// TODO Auto-generated method stub
String url = "http://www.mytheresa.com/en-de/shoes.html?allproducts=yes";
url = "http://www.mytheresa.com/en-de/clothing/dresses.html";
try{
ReturnObject<Object> rm = this.product_list(url);
if(rm!=null){
List<Object> list = rm.getRows();
for (Object object : list) {
Map<String ,String> map = (Map<String, String>) object;
System.out.println("请求的结果:"+map.get("title")+"|"+map.get("href"));
}
}
}catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
public ReturnObject<Object> product_list(String url) throws Exception{
// TODO Auto-generated method stub
System.out.println("开始抓取网址,请等候...");
System.out.println(url);
System.out.println();
ReturnObject<Object> rm = new ReturnObject<Object>();
List<Object> list = new ArrayList<Object>();
String contents = httpClient.methodGet(url);
Document doc = Jsoup.parse(contents);
Element div_category_products = doc.getElementsByAttributeValue("class", "category-products").first();
if(div_category_products == null)return null;
Elements ul = div_category_products.getElementsByTag("ul");
String href = "";
String title = "";
for (Element element_ul : ul) {
Elements product_image_a = element_ul.getElementsByAttributeValue("class","product-image");
for(Element a : product_image_a){
href = a.attr("href");
Map<String ,String> map = new HashMap<String, String>();
map.put("href", href);
list.add(map);
}
}
rm.setRows(list);
return rm;
}
@Override
@Test
public void info() {
// TODO Auto-generated method stub
String url = host + "/en-de/clothing/dresses.html";
url = "http://www.mytheresa.com/en-de/metallic-crepe-dress-356477.html";
try {
ReturnObject<Object> rm = this.product_info(url);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
public ReturnObject<Object> product_info(String url) throws Exception{
// TODO Auto-generated method stub
System.out.println("获取商品详情链接,请等候...");
System.out.println(url);
System.out.println();
ReturnObject<Object> rm = new ReturnObject<Object>();
Goods goods = new Goods();
goods.setGoodsUrl(url);
String contents = httpClient.methodGet(url);
Document doc = Jsoup.parse(contents);
Element designer_name = doc.getElementsByAttributeValue("class", "designer-name").first();
Element itemprop_brand = designer_name.getElementsByAttributeValue("itemprop", "brand").first();
String brand = itemprop_brand.html();
goods.setBrandName(brand);
Element product_name = doc.getElementsByAttributeValue("class", "product-name").first();
Element itemprop_name = product_name.getElementsByAttributeValue("itemprop", "name").first();
String name = itemprop_name.html();
goods.setGoodsName(name);
String price = doc.getElementsByAttributeValue("itemprop","price").first().attr("content");
goods.setPrice(price);
System.out.println(goods.getPrice());
// description
Element details = doc.getElementsByAttributeValue("itemprop", "description").first();
goods.setGoods_desc(details.html());
String productImage = doc.getElementById("main-image-image").attr("src");
goods.setImage(productImage);
System.out.println(productImage);
//产品id
String product = doc.getElementsByAttributeValue("name", "product").first().attr("value");
System.out.println(product);
// String num = this.regx_number(url);
//获取尺寸的html
// String sizeContainerHtml = httpClient.methodGet("http://www.mytheresa.com/en-de/mzcatalog/ajax/ajaxcartcode/id/"+product+"/");
// Document sizeDoc = Jsoup.parse(sizeContainerHtml);
//
// //尺寸
// Element sizeContainer = sizeDoc.getElementsByAttributeValue("class", "sizes").first();
Element sizeContainer = doc.getElementsByAttributeValue("class","size-chooser").first();
if(sizeContainer != null){
List<String> map_sizeContainer = this.get_attribute_sizeContainer_list(sizeContainer);
JSONArray sizeObj = JSONArray.fromObject(map_sizeContainer);
System.out.println(sizeObj.toString());
goods.setSizeJson(sizeObj.toString());
}
//相册
Element scroller_thumbs = doc.getElementById("scroller-thumbs");
List<String> goodsGallery = this.get_goods_gallery_list(scroller_thumbs);
goods.setGoodsImage(goodsGallery);
//分类
Element CategoryEl = doc.getElementsByAttributeValue("class", "breadcrumbs").first();
String category = this.get_Category(CategoryEl);
goods.setCategoryName(category);
category = null;
CategoryEl = null;
//商品编号
Element goods_sn = doc.getElementsByAttributeValue("class", "sku-number").first();
goods.setGoodsSN(goods_sn.text());
System.out.println("goods_sn:"+goods.getGoodsSN());
rm.setModel(goods);
this.PrintlnGoods(goods);
return rm;
}
@Override
public Map<String, String> get_attribute_swatches(Element swatches) {
// TODO Auto-generated method stub
return null;
}
@Override
public Map<String, String> get_attribute_sizeContainer(Element sizeContainer) {
// TODO Auto-generated method stub
//
return null;
}
@Override
public String get_next_link(String href) {
// TODO Auto-generated method stub
return null;
}
@Override
public List<String> get_attribute_swatches_list(Element swatches) {
// TODO Auto-generated method stub
return null;
}
@Override
public List<String> get_attribute_sizeContainer_list(Element sizeContainer) {
// TODO Auto-generated method stub
List<String> map_attribute_size = new ArrayList<String>();
Elements li = sizeContainer.getElementsByTag("li");
String data_selectedsize = "";
// String id = "";
Element a;
Elements span;
for (Element element : li) {
a = element.getElementsByTag("a").first();
span = a.getElementsByTag("span");
if(span.toString() == null || span.toString().equals("")){
data_selectedsize = a.html();
}else{
data_selectedsize = span.html();
}
System.out.println("data_selectedsize:"+data_selectedsize);
map_attribute_size.add( data_selectedsize);
}
return map_attribute_size;
}
public String regx_number(String str){
String regex = "\\d+.\\d+|\\w+\\d+";
String num = "";
Pattern pattern = Pattern.compile(regex);
Matcher m = pattern.matcher(str);
while(m.find()){
num = m.group();
}
return num;
}
@Override
public List<String> get_goods_gallery_list(Element goodsGallery) {
// TODO Auto-generated method stub
Elements liGG = goodsGallery.getElementsByTag("img").attr("class", "zoom-trigger");
List<String> gg = new ArrayList<String>();
String img = "";
for (Element element : liGG) {
img = element.attr("src");
if(img.indexOf("thumbnail/100x")>0){
img = img.replaceAll("thumbnail/100x", "image/420x475");
System.out.println("相册:"+img);
gg.add(img);
}
}
return gg;
}
@Override
public String get_Category(Element category) {
// TODO Auto-generated method stub
if(category == null)return "";
Elements span = category.getElementsByTag("a");
String cat_name = "";
for(Element s : span){
cat_name += s.text()+",";
}
if(cat_name.length()>0){
cat_name = cat_name.substring(0,cat_name.length() - 1);
}
System.out.println("分类:"+cat_name);
return cat_name;
}
@Override
public List<Map<String,String>> get_goods_gallery_list_Map(Element goodsGallery){
return null;
}
}
<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/model/HaiHrPosition.java
package com.ehais.hrlucene.model;
import java.io.Serializable;
public class HaiHrPosition implements Serializable {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_hr_position.position_id
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
private Long positionId;
/**
* 公司名称
*/
private String companyName;
/**
* 职位名称
*/
private String positionName;
/**
* 工作城市
*/
private String workcity;
/**
* 工作性质:全职、兼职
*/
private String fulltime;
/**
* 职位类型
*/
private String postCategory;
/**
* 行业要求
*/
private String industry;
/**
* 招聘人数
*/
private String headcount;
/**
* 学历要求
*/
private String degreelevel;
/**
* 招聘部门
*/
private String department;
/**
* 性别要求
*/
private String gender;
/**
* 工作经验
*/
private String workyears;
/**
* 年龄要求
*/
private String workages;
/**
* 联系手机号
*/
private String phone;
/**
* 联系电话
*/
private String tel;
/**
* 联系人
*/
private String contactperson;
/**
* 公司传真
*/
private String companyFax;
/**
* 公司邮箱
*/
private String companyEmail;
/**
* 公司地址
*/
private String companyAddress;
/**
* 邮政编码
*/
private String companyZip;
/**
* 薪水要求
*/
private String salary;
/**
* 婚姻要求
*/
private String marriage;
/**
* 主修课程要求
*/
private String major;
/**
* 专业要求
*/
private String profession;
/**
* 语言要求
*/
private String language;
/**
* 面试地点
*/
private String interviewplace;
/**
* 面试时间
*/
private String interviewtime;
/**
* 发布时间,即有效时间段
*/
private String issueTime;
/**
* 身高要求
*/
private String heightbody;
/**
* 户籍要求
*/
private String census;
/**
* 职称要求
*/
private String technicalpost;
/**
* 公司福利
*/
private String welfare;
/**
* 公司性质
*/
private String companyNature;
/**
* 公司规模
*/
private String companyScale;
/**
* 公司所属行业
*/
private String companyIndustry;
/**
* 公司网址
*/
private String companyWebsite;
/**
* 来源哪个人才网,中文存储可以
*/
private String hrSource;
/**
* 职位网址
*/
private String hrPositionUrl;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
private static final long serialVersionUID = 1L;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.position_id
*
* @return the value of hai_hr_position.position_id
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public Long getPositionId() {
return positionId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.position_id
*
* @param positionId the value for hai_hr_position.position_id
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setPositionId(Long positionId) {
this.positionId = positionId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.company_name
*
* @return the value of hai_hr_position.company_name
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getCompanyName() {
return companyName;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.company_name
*
* @param companyName the value for hai_hr_position.company_name
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setCompanyName(String companyName) {
this.companyName = companyName;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.position_name
*
* @return the value of hai_hr_position.position_name
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getPositionName() {
return positionName;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.position_name
*
* @param positionName the value for hai_hr_position.position_name
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setPositionName(String positionName) {
this.positionName = positionName;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.workcity
*
* @return the value of hai_hr_position.workcity
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getWorkcity() {
return workcity;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.workcity
*
* @param workcity the value for hai_hr_position.workcity
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setWorkcity(String workcity) {
this.workcity = workcity;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.fulltime
*
* @return the value of hai_hr_position.fulltime
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getFulltime() {
return fulltime;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.fulltime
*
* @param fulltime the value for hai_hr_position.fulltime
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setFulltime(String fulltime) {
this.fulltime = fulltime;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.post_category
*
* @return the value of hai_hr_position.post_category
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getPostCategory() {
return postCategory;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.post_category
*
* @param postCategory the value for hai_hr_position.post_category
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setPostCategory(String postCategory) {
this.postCategory = postCategory;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.industry
*
* @return the value of hai_hr_position.industry
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getIndustry() {
return industry;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.industry
*
* @param industry the value for hai_hr_position.industry
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setIndustry(String industry) {
this.industry = industry;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.headcount
*
* @return the value of hai_hr_position.headcount
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getHeadcount() {
return headcount;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.headcount
*
* @param headcount the value for hai_hr_position.headcount
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setHeadcount(String headcount) {
this.headcount = headcount;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.degreelevel
*
* @return the value of hai_hr_position.degreelevel
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getDegreelevel() {
return degreelevel;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.degreelevel
*
* @param degreelevel the value for hai_hr_position.degreelevel
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setDegreelevel(String degreelevel) {
this.degreelevel = degreelevel;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.department
*
* @return the value of hai_hr_position.department
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getDepartment() {
return department;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.department
*
* @param department the value for hai_hr_position.department
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setDepartment(String department) {
this.department = department;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.gender
*
* @return the value of hai_hr_position.gender
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getGender() {
return gender;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.gender
*
* @param gender the value for hai_hr_position.gender
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setGender(String gender) {
this.gender = gender;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.workyears
*
* @return the value of hai_hr_position.workyears
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getWorkyears() {
return workyears;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.workyears
*
* @param workyears the value for hai_hr_position.workyears
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setWorkyears(String workyears) {
this.workyears = workyears;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.workages
*
* @return the value of hai_hr_position.workages
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getWorkages() {
return workages;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.workages
*
* @param workages the value for hai_hr_position.workages
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setWorkages(String workages) {
this.workages = workages;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.phone
*
* @return the value of hai_hr_position.phone
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getPhone() {
return phone;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.phone
*
* @param phone the value for hai_hr_position.phone
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setPhone(String phone) {
this.phone = phone;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.tel
*
* @return the value of hai_hr_position.tel
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getTel() {
return tel;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.tel
*
* @param tel the value for hai_hr_position.tel
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setTel(String tel) {
this.tel = tel;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.contactperson
*
* @return the value of hai_hr_position.contactperson
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getContactperson() {
return contactperson;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.contactperson
*
* @param contactperson the value for hai_hr_position.contactperson
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setContactperson(String contactperson) {
this.contactperson = contactperson;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.company_fax
*
* @return the value of hai_hr_position.company_fax
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getCompanyFax() {
return companyFax;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.company_fax
*
* @param companyFax the value for hai_hr_position.company_fax
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setCompanyFax(String companyFax) {
this.companyFax = companyFax;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.company_email
*
* @return the value of hai_hr_position.company_email
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getCompanyEmail() {
return companyEmail;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.company_email
*
* @param companyEmail the value for hai_hr_position.company_email
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setCompanyEmail(String companyEmail) {
this.companyEmail = companyEmail;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.company_address
*
* @return the value of hai_hr_position.company_address
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getCompanyAddress() {
return companyAddress;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.company_address
*
* @param companyAddress the value for hai_hr_position.company_address
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setCompanyAddress(String companyAddress) {
this.companyAddress = companyAddress;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.company_zip
*
* @return the value of hai_hr_position.company_zip
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getCompanyZip() {
return companyZip;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.company_zip
*
* @param companyZip the value for hai_hr_position.company_zip
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setCompanyZip(String companyZip) {
this.companyZip = companyZip;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.marriage
*
* @return the value of hai_hr_position.marriage
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getMarriage() {
return marriage;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.marriage
*
* @param marriage the value for hai_hr_position.marriage
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setMarriage(String marriage) {
this.marriage = marriage;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.major
*
* @return the value of hai_hr_position.major
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getMajor() {
return major;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.major
*
* @param major the value for hai_hr_position.major
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setMajor(String major) {
this.major = major;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.profession
*
* @return the value of hai_hr_position.profession
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getProfession() {
return profession;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.profession
*
* @param profession the value for hai_hr_position.profession
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setProfession(String profession) {
this.profession = profession;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.language
*
* @return the value of hai_hr_position.language
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getLanguage() {
return language;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.language
*
* @param language the value for hai_hr_position.language
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setLanguage(String language) {
this.language = language;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.interviewplace
*
* @return the value of hai_hr_position.interviewplace
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getInterviewplace() {
return interviewplace;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.interviewplace
*
* @param interviewplace the value for hai_hr_position.interviewplace
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setInterviewplace(String interviewplace) {
this.interviewplace = interviewplace;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.interviewtime
*
* @return the value of hai_hr_position.interviewtime
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getInterviewtime() {
return interviewtime;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.interviewtime
*
* @param interviewtime the value for hai_hr_position.interviewtime
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setInterviewtime(String interviewtime) {
this.interviewtime = interviewtime;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.issue_time
*
* @return the value of hai_hr_position.issue_time
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getIssueTime() {
return issueTime;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.issue_time
*
* @param issueTime the value for hai_hr_position.issue_time
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setIssueTime(String issueTime) {
this.issueTime = issueTime;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.heightbody
*
* @return the value of hai_hr_position.heightbody
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getHeightbody() {
return heightbody;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.heightbody
*
* @param heightbody the value for hai_hr_position.heightbody
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setHeightbody(String heightbody) {
this.heightbody = heightbody;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.census
*
* @return the value of hai_hr_position.census
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getCensus() {
return census;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.census
*
* @param census the value for hai_hr_position.census
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setCensus(String census) {
this.census = census;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.technicalpost
*
* @return the value of hai_hr_position.technicalpost
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getTechnicalpost() {
return technicalpost;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.technicalpost
*
* @param technicalpost the value for hai_hr_position.technicalpost
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setTechnicalpost(String technicalpost) {
this.technicalpost = technicalpost;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.welfare
*
* @return the value of hai_hr_position.welfare
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getWelfare() {
return welfare;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.welfare
*
* @param welfare the value for hai_hr_position.welfare
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setWelfare(String welfare) {
this.welfare = welfare;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.company_nature
*
* @return the value of hai_hr_position.company_nature
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getCompanyNature() {
return companyNature;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.company_nature
*
* @param companyNature the value for hai_hr_position.company_nature
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setCompanyNature(String companyNature) {
this.companyNature = companyNature;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.company_scale
*
* @return the value of hai_hr_position.company_scale
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getCompanyScale() {
return companyScale;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.company_scale
*
* @param companyScale the value for hai_hr_position.company_scale
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setCompanyScale(String companyScale) {
this.companyScale = companyScale;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.company_industry
*
* @return the value of hai_hr_position.company_industry
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getCompanyIndustry() {
return companyIndustry;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.company_industry
*
* @param companyIndustry the value for hai_hr_position.company_industry
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setCompanyIndustry(String companyIndustry) {
this.companyIndustry = companyIndustry;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.company_website
*
* @return the value of hai_hr_position.company_website
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getCompanyWebsite() {
return companyWebsite;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.company_website
*
* @param companyWebsite the value for hai_hr_position.company_website
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setCompanyWebsite(String companyWebsite) {
this.companyWebsite = companyWebsite;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.hr_source
*
* @return the value of hai_hr_position.hr_source
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getHrSource() {
return hrSource;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.hr_source
*
* @param hrSource the value for hai_hr_position.hr_source
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setHrSource(String hrSource) {
this.hrSource = hrSource;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_hr_position.hr_position_url
*
* @return the value of hai_hr_position.hr_position_url
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public String getHrPositionUrl() {
return hrPositionUrl;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_hr_position.hr_position_url
*
* @param hrPositionUrl the value for hai_hr_position.hr_position_url
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
public void setHrPositionUrl(String hrPositionUrl) {
this.hrPositionUrl = hrPositionUrl;
}
public String getSalary() {
return salary;
}
public void setSalary(String salary) {
this.salary = salary;
}
}<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/ShopbopController.java
package com.ehais.figoarticle.controller;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.model.HaiCategory;
import com.ehais.figoarticle.model.HaiCategoryExample;
import com.ehais.figoarticle.model.HaiGoodsAttr;
import com.ehais.figoarticle.model.HaiGoodsEntity;
import com.ehais.figoarticle.model.HaiGoodsGallery;
import com.ehais.figoarticle.model.HaiGoodsUrl;
import com.ehais.figoarticle.model.HaiGoodsUrlExample;
import com.ehais.figoarticle.model.HaiGoodsWithBLOBs;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
//完成
@Controller
@RequestMapping("/shopbop")
public class ShopbopController extends FigoCommonController{
private static String url = "https://www.shopbop.com";
private int websiteId = 17;
@ResponseBody
@RequestMapping("/brand")
public String brand(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
return "";
}
@ResponseBody
@RequestMapping("/category")
public String category(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
this.category(request, url);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private void category(HttpServletRequest request,String categoryUrl){
String result = "";
try {
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), categoryUrl);
// result = PythonUtil.python("D:/workspace_jee/figoarticle/src/main/webapp/getAjaxWeb.py", categoryUrl);
// result = FSO.ReadFileName("C:\\Users\\wugang\\Desktop\\shopbop.html");
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(categoryUrl).timeout(10000).get();
List<HaiCategory> list = new ArrayList<HaiCategory>();
Elements navCategory = doc.select(".navCategory");
navCategory.remove(10);
navCategory.remove(9);
for (Element element : navCategory) {
Element parent = element.select(".parent").first();
System.out.println(parent.text());
String pHref = parent.attr("href");
if (pHref.indexOf("http") < 0 ) pHref = url + pHref;
HaiCategory pCate = new HaiCategory();
pCate.setCatName(parent.text());
pCate.setCategoryUrl(pHref);
pCate.setIsShow(true);
pCate.setWebsiteId(websiteId);
List<HaiCategory> children = new ArrayList<>();
Elements cates = element.select("ul>li");
for (Element element1 : cates) {
String cName = element1.text();
if (cName==null || cName.equals("")) cName="本周热卖";
System.out.println("===" + cName );
String cHref = element1.select("a").attr("href");
if (cHref.indexOf("http") < 0 ) cHref = url + cHref;
HaiCategory cCate = new HaiCategory();
cCate.setCatName(cName);
cCate.setCategoryUrl(cHref);
cCate.setIsShow(true);
cCate.setWebsiteId(websiteId);
children.add(cCate);
}
pCate.setChildren(children);
list.add(pCate);
}
System.out.println("==========================================");
System.out.println("==========================================");
JSONArray arr = JSONArray.fromObject(list);
System.out.println(arr.toString());
// System.out.println(element.html());
System.out.println("==========================================");
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/category";
// String api = "http://localhost:8087/api/category";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
}
@ResponseBody
@RequestMapping("/url")
public String url(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
c.andCategoryUrlLike(url+"%");
List<HaiCategory> listCategory = haiCategoryMapper.selectByExample(example);
for (HaiCategory haiCategory : listCategory) {
// System.out.println(haiCategory.getCategoryUrl());
this.goodsUrl(request, haiCategory.getCategoryUrl(), haiCategory.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsUrl(HttpServletRequest request,String goodsurl,Integer catId){
System.out.println("请求地址:" + goodsurl);
String result = "";
try{
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
// result = PythonUtil.python("D:/workspace_jee/figoarticle/src/main/webapp/getAjaxWeb.py", categoryUrl);
// result = FSO.ReadFileName("E:/temp/IFCHIC.htm");
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(goodsurl).timeout(10000).get();
Element productList = doc.getElementById("product-container");
if (productList == null || productList.equals("")) return "";
Elements product_li = productList.select("li");
List<String> list = new ArrayList<String>();
for (Element element : product_li) {
String Href = element.select("a").first().attr("href");
if (Href.indexOf("http") < 0) Href = url + Href;
System.out.println(Href);
list.add(Href);
}
JSONArray arr = JSONArray.fromObject(list);
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("catId", catId.toString());
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/url";
// String api = "http://localhost:8087/api/url";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
//获取下一页
Element page = doc.getElementById("pagination-container-bottom");
Element next = page.select(".pages").select(".next").first();
if (next != null) {
String nHref = next.attr("data-next-link");
if (nHref.indexOf("http") < 0) nHref = url + nHref;
this.goodsUrl(request, nHref, catId);
}
}catch(Exception e){
e.printStackTrace();
}
return result;
}
@ResponseBody
@RequestMapping("/goodsAll")
public String goodsAll(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiGoodsUrlExample example = new HaiGoodsUrlExample();
HaiGoodsUrlExample.Criteria c = example.createCriteria();
c.andGoodsUrlLike(url+"%");
c.andFinishEqualTo(false);
List<HaiGoodsUrl> listGoodsUrl = haiGoodsUrlMapper.selectByExample(example);
for (HaiGoodsUrl haiGoodsUrl : listGoodsUrl) {
goodsModel(request,haiGoodsUrl.getGoodsUrl(),haiGoodsUrl.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
public String goodsModel(HttpServletRequest request , String goodsurl ,Integer catId){
System.out.println(goodsurl);
String result = "";
HaiGoodsEntity entity = new HaiGoodsEntity();
HaiGoodsWithBLOBs goods = new HaiGoodsWithBLOBs();
List<HaiGoodsGallery> goodsGalleryList = new ArrayList<HaiGoodsGallery>();
List<HaiGoodsAttr> goodsAttrList = new ArrayList<HaiGoodsAttr>();
try{
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(goodsurl).timeout(10000).get();
Element info = doc.getElementById("product-information");
String name = info.select(".brand-heading").text();
Elements priceL = doc.getElementById("productPrices").select(".priceBlock");
String priceS = "";
if (priceL.size() == 1) {
priceS = priceL.first().text();
} else if (priceL.select(".priceColors").size() == 1 && priceL.select(".salePrice.red-text").isEmpty()) {
priceS = priceL.get(1).select(".salePrice").get(0).text();
} else if (priceL.select(".priceColors").size() == 1) {
priceS = priceL.get(1).select(".salePrice").get(1).text();
}
priceS = priceS.replace(",","");
if ( priceS != "") {
Integer price = Float.valueOf(priceS.substring(4)).intValue() * 100;
goods.setShopPrice(price);
}
String desc = doc.select("[itemprop=description]").text();
String currency = doc.select(".currency-code").first().text();
goods.setGoodsName(name);
goods.setGoodsDesc(desc);
goods.setCurrency(currency);
goods.setGoodsUrl(goodsurl);
goods.setCatId(catId);
goods.setWebsiteId(websiteId);
//picture
Element gallery_ul = doc.getElementById("thumbnailList");
Elements gallery_li = gallery_ul.select("li.thumbnailListItem");
for (Element element : gallery_li) {
String gHref = element.attr("src");
if (gHref.indexOf("http") < 0) gHref = url + gHref;
HaiGoodsGallery gallery = new HaiGoodsGallery();
gallery.setThumbUrl(gHref);
gallery.setImgUrl(gHref);
gallery.setImgOriginal(gHref);
goodsGalleryList.add(gallery);
}
HaiGoodsGallery gallery = goodsGalleryList.get(0);
goods.setGoodsThumb(gallery.getThumbUrl());
goods.setGoodsImg(gallery.getImgUrl());
goods.setOriginalImg(gallery.getImgOriginal());
//color
Element color = doc.getElementById("swatches");
Elements colorLi = color.select("img");
List<String> colorList = new ArrayList<>();
if (goods.getShopPrice() != null) {
for (Element element : colorLi) {
HaiGoodsAttr goodsColor = new HaiGoodsAttr();
goodsColor.setAttrValue(element.attr("title"));
goodsColor.setAttrType("color");
goodsColor.setAttrPrice(goods.getShopPrice().toString());
colorList.add(goodsColor.getAttrValue());
goodsAttrList.add(goodsColor);
}
}else{
Elements colorSale = doc.select(".priceBlock");
colorSale.remove(0);
for (Element element : colorSale) {
HaiGoodsAttr goodsColor = new HaiGoodsAttr();
Elements ePrice = element.select(".salePrice");
String sPrice = "";
if (ePrice.size() == 1) {
sPrice = ePrice.text();
}else {
sPrice = ePrice.get(1).text();
}
Integer cPrice = Float.valueOf(sPrice.substring(4)).intValue() * 100;
String colorName = element.select(".priceColors").text();
goodsColor.setAttrType("color");
goodsColor.setAttrValue(colorName);
goodsColor.setAttrPrice(cPrice.toString());
colorList.add(colorName);
goodsAttrList.add(goodsColor);
}
goods.setShopPrice(Float.valueOf(goodsAttrList.get(0).getAttrPrice()).intValue());
}
//size
Element size = doc.getElementById("sizes");
Elements sizes = size.select("span");
List<String> sizeList = new ArrayList<>();
for (Element element : sizes) {
HaiGoodsAttr goodsSize = new HaiGoodsAttr();
goodsSize.setAttrValue(element.text());
goodsSize.setAttrType("size");
goodsSize.setAttrPrice(goods.getShopPrice().toString());
sizeList.add(element.text());
goodsAttrList.add(goodsSize);
}
// Bean2Utils.printEntity(goods);
entity.setGoods(goods);
entity.setGoodsAttrList(goodsAttrList);
entity.setGoodsGalleryList(goodsGalleryList);
JSONObject jsonObject = JSONObject.fromObject(entity);
System.out.println(jsonObject.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", jsonObject.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/goodsAttr";
// String api = "http://localhost:8087/api/goods";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
//单商品的地址请求
@ResponseBody
@RequestMapping("/getGoodsUrl")
public String getGoodsUrl(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "catId", required = true) Integer catId,
@RequestParam(value = "goodsurl", required = true) String goodsurl
){
try{
return goodsModel(request,goodsurl,catId);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
public static void main(String[] args) throws Exception {
String goodsurl = "https://www.net-a-porter.com/cn/zh/d/Shop/Lingerie/All?cm_sp=topnav-_-clothing-_-lingerie";
DemoController ac = new DemoController();
// ac.goodsModel(url,1);
// ac.goodsUrl(null, goodsurl, 1);
}
}
<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/mapper/HaiHrPositionMapper.java
package com.ehais.hrlucene.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.ResultMap;
import com.ehais.hrlucene.model.HaiHrPosition;
import com.ehais.hrlucene.model.HaiHrPositionExample;
import com.ehais.hrlucene.model.HaiHrPositionWithBLOBs;
public interface HaiHrPositionMapper {
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
@ResultMap(value = "BaseResultMap")
List<HaiHrPosition> hai_hr_position_list(@Param("store_id") Integer store_id, @Param("start") Integer start, @Param("len") Integer len);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
@ResultMap(value = "BaseResultMap")
List<HaiHrPosition> hai_hr_position_list_by_example(HaiHrPositionExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
@ResultMap(value = "ResultMapWithBLOBs")
HaiHrPosition get_hai_hr_position_info(@Param("store_id") Integer store_id, @Param("position_id") Long position_id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
int countByExample(HaiHrPositionExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
int deleteByExample(HaiHrPositionExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
int deleteByPrimaryKey(Long positionId);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
int insert(HaiHrPositionWithBLOBs record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
int insertSelective(HaiHrPositionWithBLOBs record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
List<HaiHrPositionWithBLOBs> selectByExampleWithBLOBs(HaiHrPositionExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
List<HaiHrPosition> selectByExample(HaiHrPositionExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
HaiHrPositionWithBLOBs selectByPrimaryKey(Long positionId);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
int updateByExampleSelective(@Param("record") HaiHrPositionWithBLOBs record, @Param("example") HaiHrPositionExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
int updateByExampleWithBLOBs(@Param("record") HaiHrPositionWithBLOBs record, @Param("example") HaiHrPositionExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
int updateByExample(@Param("record") HaiHrPosition record, @Param("example") HaiHrPositionExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
int updateByPrimaryKeySelective(HaiHrPositionWithBLOBs record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
int updateByPrimaryKeyWithBLOBs(HaiHrPositionWithBLOBs record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_hr_position
*
* @mbggenerated Sat Nov 19 19:43:25 CST 2016
*/
int updateByPrimaryKey(HaiHrPosition record);
}<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/HaiGoodsAttrExample.java
package com.ehais.figoarticle.model;
import java.util.ArrayList;
import java.util.List;
public class HaiGoodsAttrExample {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
protected String orderByClause;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
protected boolean distinct;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
protected List<Criteria> oredCriteria;
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
public HaiGoodsAttrExample() {
oredCriteria = new ArrayList<Criteria>();
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
public String getOrderByClause() {
return orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
public boolean isDistinct() {
return distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andGoodsAttrIdIsNull() {
addCriterion("goods_attr_id is null");
return (Criteria) this;
}
public Criteria andGoodsAttrIdIsNotNull() {
addCriterion("goods_attr_id is not null");
return (Criteria) this;
}
public Criteria andGoodsAttrIdEqualTo(Integer value) {
addCriterion("goods_attr_id =", value, "goodsAttrId");
return (Criteria) this;
}
public Criteria andGoodsAttrIdNotEqualTo(Integer value) {
addCriterion("goods_attr_id <>", value, "goodsAttrId");
return (Criteria) this;
}
public Criteria andGoodsAttrIdGreaterThan(Integer value) {
addCriterion("goods_attr_id >", value, "goodsAttrId");
return (Criteria) this;
}
public Criteria andGoodsAttrIdGreaterThanOrEqualTo(Integer value) {
addCriterion("goods_attr_id >=", value, "goodsAttrId");
return (Criteria) this;
}
public Criteria andGoodsAttrIdLessThan(Integer value) {
addCriterion("goods_attr_id <", value, "goodsAttrId");
return (Criteria) this;
}
public Criteria andGoodsAttrIdLessThanOrEqualTo(Integer value) {
addCriterion("goods_attr_id <=", value, "goodsAttrId");
return (Criteria) this;
}
public Criteria andGoodsAttrIdIn(List<Integer> values) {
addCriterion("goods_attr_id in", values, "goodsAttrId");
return (Criteria) this;
}
public Criteria andGoodsAttrIdNotIn(List<Integer> values) {
addCriterion("goods_attr_id not in", values, "goodsAttrId");
return (Criteria) this;
}
public Criteria andGoodsAttrIdBetween(Integer value1, Integer value2) {
addCriterion("goods_attr_id between", value1, value2, "goodsAttrId");
return (Criteria) this;
}
public Criteria andGoodsAttrIdNotBetween(Integer value1, Integer value2) {
addCriterion("goods_attr_id not between", value1, value2, "goodsAttrId");
return (Criteria) this;
}
public Criteria andGoodsIdIsNull() {
addCriterion("goods_id is null");
return (Criteria) this;
}
public Criteria andGoodsIdIsNotNull() {
addCriterion("goods_id is not null");
return (Criteria) this;
}
public Criteria andGoodsIdEqualTo(Long value) {
addCriterion("goods_id =", value, "goodsId");
return (Criteria) this;
}
public Criteria andGoodsIdNotEqualTo(Long value) {
addCriterion("goods_id <>", value, "goodsId");
return (Criteria) this;
}
public Criteria andGoodsIdGreaterThan(Long value) {
addCriterion("goods_id >", value, "goodsId");
return (Criteria) this;
}
public Criteria andGoodsIdGreaterThanOrEqualTo(Long value) {
addCriterion("goods_id >=", value, "goodsId");
return (Criteria) this;
}
public Criteria andGoodsIdLessThan(Long value) {
addCriterion("goods_id <", value, "goodsId");
return (Criteria) this;
}
public Criteria andGoodsIdLessThanOrEqualTo(Long value) {
addCriterion("goods_id <=", value, "goodsId");
return (Criteria) this;
}
public Criteria andGoodsIdIn(List<Long> values) {
addCriterion("goods_id in", values, "goodsId");
return (Criteria) this;
}
public Criteria andGoodsIdNotIn(List<Long> values) {
addCriterion("goods_id not in", values, "goodsId");
return (Criteria) this;
}
public Criteria andGoodsIdBetween(Long value1, Long value2) {
addCriterion("goods_id between", value1, value2, "goodsId");
return (Criteria) this;
}
public Criteria andGoodsIdNotBetween(Long value1, Long value2) {
addCriterion("goods_id not between", value1, value2, "goodsId");
return (Criteria) this;
}
public Criteria andAttrPriceIsNull() {
addCriterion("attr_price is null");
return (Criteria) this;
}
public Criteria andAttrPriceIsNotNull() {
addCriterion("attr_price is not null");
return (Criteria) this;
}
public Criteria andAttrPriceEqualTo(String value) {
addCriterion("attr_price =", value, "attrPrice");
return (Criteria) this;
}
public Criteria andAttrPriceNotEqualTo(String value) {
addCriterion("attr_price <>", value, "attrPrice");
return (Criteria) this;
}
public Criteria andAttrPriceGreaterThan(String value) {
addCriterion("attr_price >", value, "attrPrice");
return (Criteria) this;
}
public Criteria andAttrPriceGreaterThanOrEqualTo(String value) {
addCriterion("attr_price >=", value, "attrPrice");
return (Criteria) this;
}
public Criteria andAttrPriceLessThan(String value) {
addCriterion("attr_price <", value, "attrPrice");
return (Criteria) this;
}
public Criteria andAttrPriceLessThanOrEqualTo(String value) {
addCriterion("attr_price <=", value, "attrPrice");
return (Criteria) this;
}
public Criteria andAttrPriceLike(String value) {
addCriterion("attr_price like", value, "attrPrice");
return (Criteria) this;
}
public Criteria andAttrPriceNotLike(String value) {
addCriterion("attr_price not like", value, "attrPrice");
return (Criteria) this;
}
public Criteria andAttrPriceIn(List<String> values) {
addCriterion("attr_price in", values, "attrPrice");
return (Criteria) this;
}
public Criteria andAttrPriceNotIn(List<String> values) {
addCriterion("attr_price not in", values, "attrPrice");
return (Criteria) this;
}
public Criteria andAttrPriceBetween(String value1, String value2) {
addCriterion("attr_price between", value1, value2, "attrPrice");
return (Criteria) this;
}
public Criteria andAttrPriceNotBetween(String value1, String value2) {
addCriterion("attr_price not between", value1, value2, "attrPrice");
return (Criteria) this;
}
public Criteria andAttrTypeIsNull() {
addCriterion("attr_type is null");
return (Criteria) this;
}
public Criteria andAttrTypeIsNotNull() {
addCriterion("attr_type is not null");
return (Criteria) this;
}
public Criteria andAttrTypeEqualTo(String value) {
addCriterion("attr_type =", value, "attrType");
return (Criteria) this;
}
public Criteria andAttrTypeNotEqualTo(String value) {
addCriterion("attr_type <>", value, "attrType");
return (Criteria) this;
}
public Criteria andAttrTypeGreaterThan(String value) {
addCriterion("attr_type >", value, "attrType");
return (Criteria) this;
}
public Criteria andAttrTypeGreaterThanOrEqualTo(String value) {
addCriterion("attr_type >=", value, "attrType");
return (Criteria) this;
}
public Criteria andAttrTypeLessThan(String value) {
addCriterion("attr_type <", value, "attrType");
return (Criteria) this;
}
public Criteria andAttrTypeLessThanOrEqualTo(String value) {
addCriterion("attr_type <=", value, "attrType");
return (Criteria) this;
}
public Criteria andAttrTypeLike(String value) {
addCriterion("attr_type like", value, "attrType");
return (Criteria) this;
}
public Criteria andAttrTypeNotLike(String value) {
addCriterion("attr_type not like", value, "attrType");
return (Criteria) this;
}
public Criteria andAttrTypeIn(List<String> values) {
addCriterion("attr_type in", values, "attrType");
return (Criteria) this;
}
public Criteria andAttrTypeNotIn(List<String> values) {
addCriterion("attr_type not in", values, "attrType");
return (Criteria) this;
}
public Criteria andAttrTypeBetween(String value1, String value2) {
addCriterion("attr_type between", value1, value2, "attrType");
return (Criteria) this;
}
public Criteria andAttrTypeNotBetween(String value1, String value2) {
addCriterion("attr_type not between", value1, value2, "attrType");
return (Criteria) this;
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table hai_goods_attr
*
* @mbg.generated do_not_delete_during_merge Sun Apr 16 21:31:22 CST 2017
*/
public static class Criteria extends GeneratedCriteria {
protected Criteria() {
super();
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table hai_goods_attr
*
* @mbg.generated Sun Apr 16 21:31:22 CST 2017
*/
public static class Criterion {
private String condition;
private Object value;
private Object secondValue;
private boolean noValue;
private boolean singleValue;
private boolean betweenValue;
private boolean listValue;
private String typeHandler;
public String getCondition() {
return condition;
}
public Object getValue() {
return value;
}
public Object getSecondValue() {
return secondValue;
}
public boolean isNoValue() {
return noValue;
}
public boolean isSingleValue() {
return singleValue;
}
public boolean isBetweenValue() {
return betweenValue;
}
public boolean isListValue() {
return listValue;
}
public String getTypeHandler() {
return typeHandler;
}
protected Criterion(String condition) {
super();
this.condition = condition;
this.typeHandler = null;
this.noValue = true;
}
protected Criterion(String condition, Object value, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.typeHandler = typeHandler;
if (value instanceof List<?>) {
this.listValue = true;
} else {
this.singleValue = true;
}
}
protected Criterion(String condition, Object value) {
this(condition, value, null);
}
protected Criterion(String condition, Object value, Object secondValue, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.secondValue = secondValue;
this.typeHandler = typeHandler;
this.betweenValue = true;
}
protected Criterion(String condition, Object value, Object secondValue) {
this(condition, value, secondValue, null);
}
}
}<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/service/HnrcService.java
package com.ehais.hrlucene.service;
public interface HnrcService {
/**
* @描述 检索湖南人才网
* @throws Exception
* @作者 oldbiwang
* @日期 2016年11月19日
* @返回 void
*/
public void loadHnrc() throws Exception;
}
<file_sep>/school-weixin/src/main/java/com/ehais/schoolweixin/service/impl/ActivityServiceImpl.java
package com.ehais.schoolweixin.service.impl;
import java.util.List;
import org.ehais.tools.ReturnObject;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.ehais.schoolweixin.mapper.activityDetailMapper;
import com.ehais.schoolweixin.mapper.activitynameMapper;
import com.ehais.schoolweixin.model.activityDetail;
import com.ehais.schoolweixin.model.activityDetailExample;
import com.ehais.schoolweixin.model.activityname;
import com.ehais.schoolweixin.model.activitynameExample;
import com.ehais.schoolweixin.service.ActivityService;
@Service("ActivityService")
public class ActivityServiceImpl implements ActivityService{
@Autowired
private activitynameMapper alMapper;
@Autowired
private activityDetailMapper adMapper;
//保存活动发起的信息
public ReturnObject<?> saveActivity(activityname al) {
//Code先设为0,作用为判断是否保存成功
ReturnObject<?> rm = new ReturnObject<>();
rm.setCode(0);
activitynameExample ale = new activitynameExample();
ale.createCriteria().andActivityNameEqualTo(al.getActivityName());
//这是数据库是否有这条记录的活动
int count = -1;
//System.out.println("test4");
try {
count = alMapper.countByExample(ale);
//System.out.println("test4");
System.out.println("count" + count);
if(count != -1 && count != 0) {
rm.setMsg("活动已发起不能重复!");
} else {
alMapper.insert(al);
rm.setCode(1);
rm.setMsg("活动发起成功!");
}
} catch(Exception e) {
e.printStackTrace();
System.out.println("数据库操作失败!");
return rm;
}
return rm;
}
//得到所有的活动信息
public ReturnObject<activityname> getActivities(){
ReturnObject<activityname> ro = new ReturnObject<>();
activitynameExample ane = new activitynameExample();
ane.createCriteria().andActivityNameIsNotNull();
try {
//获取所有的活动
List<activityname> ans = alMapper.selectByExample(ane);
if(ans != null) {
ro.setRows(ans);
ro.setCode(1);
ro.setMsg("查询成功!");
System.out.println("查询成功活动列表!");
}
} catch (Exception e) {
System.out.println("操作数据库失败!");
e.printStackTrace();
return ro;
}
return ro;
}
//通过活动名获取id
public int getId(String name) {
//返回的id值
int id=-1;
List<activityname> list;
activitynameExample ane = new activitynameExample();
ane.createCriteria().andActivityNameEqualTo(name);
try {
list = alMapper.selectByExample(ane);
System.out.println("查询成功!");
}catch(Exception e) {
System.out.println("操作数据库失败!");
e.printStackTrace();
return -1;
}
//获取活动的id值
id = list.get(0).getId();
return id;
}
//保存报名的人员
public ReturnObject<activityDetail> savedetail(activityDetail detail) {
ReturnObject<activityDetail> ro = new ReturnObject<>();
if(detail != null) {
try {
int status = adMapper.insert(detail);
System.out.println("status = " + status);
ro.setMsg("报名成功!");
ro.setCode(1);
activityname an = alMapper.selectByPrimaryKey(detail.getId());
if(an != null) {
an.setpCount(an.getpCount() + 1);
alMapper.updateByPrimaryKey(an);
}
}catch(Exception e) {
e.printStackTrace();
ro.setMsg("报名失败!");
System.out.println("报名失败!");
return ro;
}
}
activityDetailExample ex = new activityDetailExample();
ex.createCriteria().andIdEqualTo(detail.getId());
try {
List<activityDetail> detaillist = adMapper.selectByExample(ex);
ro.setRows(detaillist);
}catch(Exception e) {
e.printStackTrace();
ro.setMsg("查询人员失败!");
System.out.println("查询人员失败!");
}
return ro;
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/HaiGoodsUrlExample.java
package com.ehais.figoarticle.model;
import java.util.ArrayList;
import java.util.List;
import org.ehais.tools.CriteriaObject;
public class HaiGoodsUrlExample {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected String orderByClause;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected boolean distinct;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected List<Criteria> oredCriteria;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected int limitStart = -1;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected int limitEnd = -1;
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public HaiGoodsUrlExample() {
oredCriteria = new ArrayList<Criteria>();
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getOrderByClause() {
return orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public boolean isDistinct() {
return distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setLimitStart(int limitStart) {
this.limitStart=limitStart;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public int getLimitStart() {
return limitStart;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setLimitEnd(int limitEnd) {
this.limitEnd=limitEnd;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public int getLimitEnd() {
return limitEnd;
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andGoodsUrlIdIsNull() {
addCriterion("goods_url_id is null");
return (Criteria) this;
}
public Criteria andGoodsUrlIdIsNotNull() {
addCriterion("goods_url_id is not null");
return (Criteria) this;
}
public Criteria andGoodsUrlIdEqualTo(Integer value) {
addCriterion("goods_url_id =", value, "goodsUrlId");
return (Criteria) this;
}
public Criteria andGoodsUrlIdNotEqualTo(Integer value) {
addCriterion("goods_url_id <>", value, "goodsUrlId");
return (Criteria) this;
}
public Criteria andGoodsUrlIdGreaterThan(Integer value) {
addCriterion("goods_url_id >", value, "goodsUrlId");
return (Criteria) this;
}
public Criteria andGoodsUrlIdGreaterThanOrEqualTo(Integer value) {
addCriterion("goods_url_id >=", value, "goodsUrlId");
return (Criteria) this;
}
public Criteria andGoodsUrlIdLessThan(Integer value) {
addCriterion("goods_url_id <", value, "goodsUrlId");
return (Criteria) this;
}
public Criteria andGoodsUrlIdLessThanOrEqualTo(Integer value) {
addCriterion("goods_url_id <=", value, "goodsUrlId");
return (Criteria) this;
}
public Criteria andGoodsUrlIdIn(List<Integer> values) {
addCriterion("goods_url_id in", values, "goodsUrlId");
return (Criteria) this;
}
public Criteria andGoodsUrlIdNotIn(List<Integer> values) {
addCriterion("goods_url_id not in", values, "goodsUrlId");
return (Criteria) this;
}
public Criteria andGoodsUrlIdBetween(Integer value1, Integer value2) {
addCriterion("goods_url_id between", value1, value2, "goodsUrlId");
return (Criteria) this;
}
public Criteria andGoodsUrlIdNotBetween(Integer value1, Integer value2) {
addCriterion("goods_url_id not between", value1, value2, "goodsUrlId");
return (Criteria) this;
}
public Criteria andCatIdIsNull() {
addCriterion("cat_id is null");
return (Criteria) this;
}
public Criteria andCatIdIsNotNull() {
addCriterion("cat_id is not null");
return (Criteria) this;
}
public Criteria andCatIdEqualTo(Integer value) {
addCriterion("cat_id =", value, "catId");
return (Criteria) this;
}
public Criteria andCatIdNotEqualTo(Integer value) {
addCriterion("cat_id <>", value, "catId");
return (Criteria) this;
}
public Criteria andCatIdGreaterThan(Integer value) {
addCriterion("cat_id >", value, "catId");
return (Criteria) this;
}
public Criteria andCatIdGreaterThanOrEqualTo(Integer value) {
addCriterion("cat_id >=", value, "catId");
return (Criteria) this;
}
public Criteria andCatIdLessThan(Integer value) {
addCriterion("cat_id <", value, "catId");
return (Criteria) this;
}
public Criteria andCatIdLessThanOrEqualTo(Integer value) {
addCriterion("cat_id <=", value, "catId");
return (Criteria) this;
}
public Criteria andCatIdIn(List<Integer> values) {
addCriterion("cat_id in", values, "catId");
return (Criteria) this;
}
public Criteria andCatIdNotIn(List<Integer> values) {
addCriterion("cat_id not in", values, "catId");
return (Criteria) this;
}
public Criteria andCatIdBetween(Integer value1, Integer value2) {
addCriterion("cat_id between", value1, value2, "catId");
return (Criteria) this;
}
public Criteria andCatIdNotBetween(Integer value1, Integer value2) {
addCriterion("cat_id not between", value1, value2, "catId");
return (Criteria) this;
}
public Criteria andGoodsUrlIsNull() {
addCriterion("goods_url is null");
return (Criteria) this;
}
public Criteria andGoodsUrlIsNotNull() {
addCriterion("goods_url is not null");
return (Criteria) this;
}
public Criteria andGoodsUrlEqualTo(String value) {
addCriterion("goods_url =", value, "goodsUrl");
return (Criteria) this;
}
public Criteria andGoodsUrlNotEqualTo(String value) {
addCriterion("goods_url <>", value, "goodsUrl");
return (Criteria) this;
}
public Criteria andGoodsUrlGreaterThan(String value) {
addCriterion("goods_url >", value, "goodsUrl");
return (Criteria) this;
}
public Criteria andGoodsUrlGreaterThanOrEqualTo(String value) {
addCriterion("goods_url >=", value, "goodsUrl");
return (Criteria) this;
}
public Criteria andGoodsUrlLessThan(String value) {
addCriterion("goods_url <", value, "goodsUrl");
return (Criteria) this;
}
public Criteria andGoodsUrlLessThanOrEqualTo(String value) {
addCriterion("goods_url <=", value, "goodsUrl");
return (Criteria) this;
}
public Criteria andGoodsUrlLike(String value) {
addCriterion("goods_url like", value, "goodsUrl");
return (Criteria) this;
}
public Criteria andGoodsUrlNotLike(String value) {
addCriterion("goods_url not like", value, "goodsUrl");
return (Criteria) this;
}
public Criteria andGoodsUrlIn(List<String> values) {
addCriterion("goods_url in", values, "goodsUrl");
return (Criteria) this;
}
public Criteria andGoodsUrlNotIn(List<String> values) {
addCriterion("goods_url not in", values, "goodsUrl");
return (Criteria) this;
}
public Criteria andGoodsUrlBetween(String value1, String value2) {
addCriterion("goods_url between", value1, value2, "goodsUrl");
return (Criteria) this;
}
public Criteria andGoodsUrlNotBetween(String value1, String value2) {
addCriterion("goods_url not between", value1, value2, "goodsUrl");
return (Criteria) this;
}
public Criteria andSpiderSourceIsNull() {
addCriterion("spider_source is null");
return (Criteria) this;
}
public Criteria andSpiderSourceIsNotNull() {
addCriterion("spider_source is not null");
return (Criteria) this;
}
public Criteria andSpiderSourceEqualTo(String value) {
addCriterion("spider_source =", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceNotEqualTo(String value) {
addCriterion("spider_source <>", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceGreaterThan(String value) {
addCriterion("spider_source >", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceGreaterThanOrEqualTo(String value) {
addCriterion("spider_source >=", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceLessThan(String value) {
addCriterion("spider_source <", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceLessThanOrEqualTo(String value) {
addCriterion("spider_source <=", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceLike(String value) {
addCriterion("spider_source like", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceNotLike(String value) {
addCriterion("spider_source not like", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceIn(List<String> values) {
addCriterion("spider_source in", values, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceNotIn(List<String> values) {
addCriterion("spider_source not in", values, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceBetween(String value1, String value2) {
addCriterion("spider_source between", value1, value2, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceNotBetween(String value1, String value2) {
addCriterion("spider_source not between", value1, value2, "spiderSource");
return (Criteria) this;
}
public Criteria andFinishIsNull() {
addCriterion("finish is null");
return (Criteria) this;
}
public Criteria andFinishIsNotNull() {
addCriterion("finish is not null");
return (Criteria) this;
}
public Criteria andFinishEqualTo(Boolean value) {
addCriterion("finish =", value, "finish");
return (Criteria) this;
}
public Criteria andFinishNotEqualTo(Boolean value) {
addCriterion("finish <>", value, "finish");
return (Criteria) this;
}
public Criteria andFinishGreaterThan(Boolean value) {
addCriterion("finish >", value, "finish");
return (Criteria) this;
}
public Criteria andFinishGreaterThanOrEqualTo(Boolean value) {
addCriterion("finish >=", value, "finish");
return (Criteria) this;
}
public Criteria andFinishLessThan(Boolean value) {
addCriterion("finish <", value, "finish");
return (Criteria) this;
}
public Criteria andFinishLessThanOrEqualTo(Boolean value) {
addCriterion("finish <=", value, "finish");
return (Criteria) this;
}
public Criteria andFinishIn(List<Boolean> values) {
addCriterion("finish in", values, "finish");
return (Criteria) this;
}
public Criteria andFinishNotIn(List<Boolean> values) {
addCriterion("finish not in", values, "finish");
return (Criteria) this;
}
public Criteria andFinishBetween(Boolean value1, Boolean value2) {
addCriterion("finish between", value1, value2, "finish");
return (Criteria) this;
}
public Criteria andFinishNotBetween(Boolean value1, Boolean value2) {
addCriterion("finish not between", value1, value2, "finish");
return (Criteria) this;
}
public Criteria andGoodsUrlLikeInsensitive(String value) {
addCriterion("upper(goods_url) like", value.toUpperCase(), "goodsUrl");
return (Criteria) this;
}
public Criteria andSpiderSourceLikeInsensitive(String value) {
addCriterion("upper(spider_source) like", value.toUpperCase(), "spiderSource");
return (Criteria) this;
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table hai_goods_url
*
* @mbg.generated do_not_delete_during_merge Sun Nov 26 11:43:10 CST 2017
*/
public static class Criteria extends GeneratedCriteria {
protected Criteria() {
super();
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table hai_goods_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public static class Criterion {
private String condition;
private Object value;
private Object secondValue;
private boolean noValue;
private boolean singleValue;
private boolean betweenValue;
private boolean listValue;
private String typeHandler;
public String getCondition() {
return condition;
}
public Object getValue() {
return value;
}
public Object getSecondValue() {
return secondValue;
}
public boolean isNoValue() {
return noValue;
}
public boolean isSingleValue() {
return singleValue;
}
public boolean isBetweenValue() {
return betweenValue;
}
public boolean isListValue() {
return listValue;
}
public String getTypeHandler() {
return typeHandler;
}
protected Criterion(String condition) {
super();
this.condition = condition;
this.typeHandler = null;
this.noValue = true;
}
protected Criterion(String condition, Object value, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.typeHandler = typeHandler;
if (value instanceof List<?>) {
this.listValue = true;
} else {
this.singleValue = true;
}
}
protected Criterion(String condition, Object value) {
this(condition, value, null);
}
protected Criterion(String condition, Object value, Object secondValue, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.secondValue = secondValue;
this.typeHandler = typeHandler;
this.betweenValue = true;
}
protected Criterion(String condition, Object value, Object secondValue) {
this(condition, value, secondValue, null);
}
}
}<file_sep>/eh-common/src/main/java/org/ehais/enums/EArticleModuleEnum.java
package org.ehais.enums;
public class EArticleModuleEnum {
public final static String INFORMATEION = "information";//资讯新闻
public final static String ACTIVITY = "activity";//资讯新闻
public final static String INDUSTRY = "industry";//行业动态
public final static String JOB = "job";//招聘信息
public final static String ABOUT = "about";//关于我们
}
<file_sep>/eh-common/src/main/java/org/ehais/mapper/WxUsersMapper.java
package org.ehais.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import org.ehais.model.WxUsers;
import org.ehais.model.WxUsersExample;
public interface WxUsersMapper {
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
long countByExample(WxUsersExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
int deleteByExample(WxUsersExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
int deleteByPrimaryKey(Integer user_id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
int insert(WxUsers record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
int insertSelective(WxUsers record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
List<WxUsers> selectByExampleWithBLOBs(WxUsersExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
List<WxUsers> selectByExample(WxUsersExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
WxUsers selectByPrimaryKey(Integer user_id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
int updateByExampleSelective(@Param("record") WxUsers record, @Param("example") WxUsersExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
int updateByExampleWithBLOBs(@Param("record") WxUsers record, @Param("example") WxUsersExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
int updateByExample(@Param("record") WxUsers record, @Param("example") WxUsersExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
int updateByPrimaryKeySelective(WxUsers record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
int updateByPrimaryKeyWithBLOBs(WxUsers record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
int updateByPrimaryKey(WxUsers record);
}<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/App.java
package com.ehais.hrlucene;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.ehais.hrlucene.service.FjrcService;
import com.ehais.hrlucene.service.impl.GgxhService;
/**
* Hello world!
*
*/
public class App
{
public static void main( String[] args ) throws Exception
{
System.out.println( "Hello World!" );
// ApplicationContext context = new FileSystemXmlApplicationContext(new String[] {"classpath*:appContext.xml","classpath*:appContext-jdbc.xml"});
ApplicationContext context = new ClassPathXmlApplicationContext("spring/appContext.xml");
// ApplicationContext context = new ClassPathXmlApplicationContext("classpath*:spring/appContext.xml");
// ApplicationContext context = new ClassPathXmlApplicationContext(new String[] {"appContext.xml","appContext-jdbc.xml"});
// GxrcService gxrcService = (GxrcService)context.getBean("gxrcService");
// gxrcService.loadGxrc();
//GzrcService gzrcService=(GzrcService)context.getBean("GzrcService");
//gzrcService.loadGzrc();
GgxhService ggxhService=(GgxhService)context.getBean("GgxhService");
ggxhService.mainScrape();
// ScrcService scrcService=(ScrcService)context.getBean("ScrcService");
// scrcService.Loadrc(null);
//ScrcService scrcService=(ScrcService)context.getBean("ScrcService");
//scrcService.Loadrc();
// FjrcService fjrcService = (FjrcService)context.getBean("FjrcService");
// fjrcService.LoadFjrc();
// SpringTaskService springTaskService = (SpringTaskService)context.getBean("springTaskService");
//
// springTaskService.myTask();
// HaiNanrcService service = (HaiNanrcService)context.getBean("hainanrcService");
// service.loadHaiNanrc();
// HnrcService service = (HnrcService)context.getBean("hnrcService");
// service.loadHnrc();
// YnrcService serivce = (YnrcService)context.getBean("ynrcService");
// serivce.loadGxrc();
}
}
<file_sep>/reptileV1/src/main/java/com/ehais/bean/Goods.java
package com.ehais.bean;
import java.util.List;
import java.util.Map;
public class Goods {
private Integer goodsId;
private String goodsName;
private String actDesc;
private String goodsSN;
private String categoryName;
private String brandName;
private String colorJson;
private String sizeJson;
private String image;
private String price;
private String market_price;
private String shop_price;
private String promote_price;
private String cost_price;
private String content;
private String goods_desc;
private String currency;
private String exchangeRate;
private String profit;
private String profitType;
private String shipping;
private String goodsUrl;//商品连接页面
private String storeId;
private List<String> thumbImage;//缩略图,首页或列表页显示的图片
private List<String> goodsImage;//详情页显示的图片
private List<String> originalImage;//源图
private String goods_thumb;
private String goods_img;
private String original_img;
/**
* 相册
* Map.put("thumb","");
* Map.put("img","");
* Map.put("original","");
*/
public List<Map<String,String>> goods_gallery;
public Goods() {
// TODO Auto-generated constructor stub
}
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
}
public Integer getGoodsId() {
return goodsId;
}
public void setGoodsId(Integer goodsId) {
this.goodsId = goodsId;
}
public String getGoodsName() {
return goodsName;
}
public void setGoodsName(String goodsName) {
this.goodsName = goodsName;
}
public String getBrandName() {
return brandName;
}
public void setBrandName(String brandName) {
this.brandName = brandName;
}
public String getColorJson() {
return colorJson;
}
public void setColorJson(String colorJson) {
this.colorJson = colorJson;
}
public String getSizeJson() {
return sizeJson;
}
public void setSizeJson(String sizeJson) {
this.sizeJson = sizeJson;
}
public String getCategoryName() {
return categoryName;
}
public void setCategoryName(String categoryName) {
this.categoryName = categoryName;
}
public String getImage() {
return image;
}
public void setImage(String image) {
this.image = image;
}
public String getPrice() {
return price;
}
public void setPrice(String price) {
this.price = price;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public String getExchangeRate() {
return exchangeRate;
}
public void setExchangeRate(String exchangeRate) {
this.exchangeRate = exchangeRate;
}
public String getProfit() {
return profit;
}
public void setProfit(String profit) {
this.profit = profit;
}
public String getProfitType() {
return profitType;
}
public void setProfitType(String profitType) {
this.profitType = profitType;
}
public String getShipping() {
return shipping;
}
public void setShipping(String shipping) {
this.shipping = shipping;
}
public String getGoodsUrl() {
return goodsUrl;
}
public void setGoodsUrl(String goodsUrl) {
this.goodsUrl = goodsUrl;
}
public List<String> getThumbImage() {
return thumbImage;
}
public void setThumbImage(List<String> thumbImage) {
this.thumbImage = thumbImage;
}
public List<String> getGoodsImage() {
return goodsImage;
}
public void setGoodsImage(List<String> goodsImage) {
this.goodsImage = goodsImage;
}
public List<String> getOriginalImage() {
return originalImage;
}
public void setOriginalImage(List<String> originalImage) {
this.originalImage = originalImage;
}
public String getStoreId() {
return storeId;
}
public void setStoreId(String storeId) {
this.storeId = storeId;
}
public String getGoodsSN() {
return goodsSN;
}
public void setGoodsSN(String goodsSN) {
this.goodsSN = goodsSN;
}
public String getActDesc() {
return actDesc;
}
public void setActDesc(String actDesc) {
this.actDesc = actDesc;
}
public String getGoods_thumb() {
return goods_thumb;
}
public void setGoods_thumb(String goods_thumb) {
this.goods_thumb = goods_thumb;
}
public String getGoods_img() {
return goods_img;
}
public void setGoods_img(String goods_img) {
this.goods_img = goods_img;
}
public String getOriginal_img() {
return original_img;
}
public void setOriginal_img(String original_img) {
this.original_img = original_img;
}
public List<Map<String, String>> getGoods_gallery() {
return goods_gallery;
}
public void setGoods_gallery(List<Map<String, String>> goods_gallery) {
this.goods_gallery = goods_gallery;
}
public String getMarket_price() {
return market_price;
}
public void setMarket_price(String market_price) {
this.market_price = market_price;
}
public String getShop_price() {
return shop_price;
}
public void setShop_price(String shop_price) {
this.shop_price = shop_price;
}
public String getPromote_price() {
return promote_price;
}
public void setPromote_price(String promote_price) {
this.promote_price = promote_price;
}
public String getGoods_desc() {
return goods_desc;
}
public void setGoods_desc(String goods_desc) {
this.goods_desc = goods_desc;
}
public String getCost_price() {
return cost_price;
}
public void setCost_price(String cost_price) {
this.cost_price = cost_price;
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/WebSiteCount.java
package com.ehais.figoarticle.model;
import java.io.Serializable;
public class WebSiteCount implements Serializable{
public Integer count;
public Integer websiteId;
public Integer getCount() {
return count;
}
public void setCount(Integer count) {
this.count = count;
}
public Integer getWebsiteId() {
return websiteId;
}
public void setWebsiteId(Integer websiteId) {
this.websiteId = websiteId;
}
}
<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/model/HaiArticleExample.java
package com.ehais.hrlucene.model;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class HaiArticleExample {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
protected String orderByClause;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
protected boolean distinct;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
protected List<Criteria> oredCriteria;
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public HaiArticleExample() {
oredCriteria = new ArrayList<Criteria>();
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public String getOrderByClause() {
return orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public boolean isDistinct() {
return distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andArticle_idIsNull() {
addCriterion("article_id is null");
return (Criteria) this;
}
public Criteria andArticle_idIsNotNull() {
addCriterion("article_id is not null");
return (Criteria) this;
}
public Criteria andArticle_idEqualTo(Integer value) {
addCriterion("article_id =", value, "article_id");
return (Criteria) this;
}
public Criteria andArticle_idNotEqualTo(Integer value) {
addCriterion("article_id <>", value, "article_id");
return (Criteria) this;
}
public Criteria andArticle_idGreaterThan(Integer value) {
addCriterion("article_id >", value, "article_id");
return (Criteria) this;
}
public Criteria andArticle_idGreaterThanOrEqualTo(Integer value) {
addCriterion("article_id >=", value, "article_id");
return (Criteria) this;
}
public Criteria andArticle_idLessThan(Integer value) {
addCriterion("article_id <", value, "article_id");
return (Criteria) this;
}
public Criteria andArticle_idLessThanOrEqualTo(Integer value) {
addCriterion("article_id <=", value, "article_id");
return (Criteria) this;
}
public Criteria andArticle_idIn(List<Integer> values) {
addCriterion("article_id in", values, "article_id");
return (Criteria) this;
}
public Criteria andArticle_idNotIn(List<Integer> values) {
addCriterion("article_id not in", values, "article_id");
return (Criteria) this;
}
public Criteria andArticle_idBetween(Integer value1, Integer value2) {
addCriterion("article_id between", value1, value2, "article_id");
return (Criteria) this;
}
public Criteria andArticle_idNotBetween(Integer value1, Integer value2) {
addCriterion("article_id not between", value1, value2, "article_id");
return (Criteria) this;
}
public Criteria andCat_idIsNull() {
addCriterion("cat_id is null");
return (Criteria) this;
}
public Criteria andCat_idIsNotNull() {
addCriterion("cat_id is not null");
return (Criteria) this;
}
public Criteria andCat_idEqualTo(Integer value) {
addCriterion("cat_id =", value, "cat_id");
return (Criteria) this;
}
public Criteria andCat_idNotEqualTo(Integer value) {
addCriterion("cat_id <>", value, "cat_id");
return (Criteria) this;
}
public Criteria andCat_idGreaterThan(Integer value) {
addCriterion("cat_id >", value, "cat_id");
return (Criteria) this;
}
public Criteria andCat_idGreaterThanOrEqualTo(Integer value) {
addCriterion("cat_id >=", value, "cat_id");
return (Criteria) this;
}
public Criteria andCat_idLessThan(Integer value) {
addCriterion("cat_id <", value, "cat_id");
return (Criteria) this;
}
public Criteria andCat_idLessThanOrEqualTo(Integer value) {
addCriterion("cat_id <=", value, "cat_id");
return (Criteria) this;
}
public Criteria andCat_idIn(List<Integer> values) {
addCriterion("cat_id in", values, "cat_id");
return (Criteria) this;
}
public Criteria andCat_idNotIn(List<Integer> values) {
addCriterion("cat_id not in", values, "cat_id");
return (Criteria) this;
}
public Criteria andCat_idBetween(Integer value1, Integer value2) {
addCriterion("cat_id between", value1, value2, "cat_id");
return (Criteria) this;
}
public Criteria andCat_idNotBetween(Integer value1, Integer value2) {
addCriterion("cat_id not between", value1, value2, "cat_id");
return (Criteria) this;
}
public Criteria andCodeIsNull() {
addCriterion("code is null");
return (Criteria) this;
}
public Criteria andCodeIsNotNull() {
addCriterion("code is not null");
return (Criteria) this;
}
public Criteria andCodeEqualTo(String value) {
addCriterion("code =", value, "code");
return (Criteria) this;
}
public Criteria andCodeNotEqualTo(String value) {
addCriterion("code <>", value, "code");
return (Criteria) this;
}
public Criteria andCodeGreaterThan(String value) {
addCriterion("code >", value, "code");
return (Criteria) this;
}
public Criteria andCodeGreaterThanOrEqualTo(String value) {
addCriterion("code >=", value, "code");
return (Criteria) this;
}
public Criteria andCodeLessThan(String value) {
addCriterion("code <", value, "code");
return (Criteria) this;
}
public Criteria andCodeLessThanOrEqualTo(String value) {
addCriterion("code <=", value, "code");
return (Criteria) this;
}
public Criteria andCodeLike(String value) {
addCriterion("code like", value, "code");
return (Criteria) this;
}
public Criteria andCodeNotLike(String value) {
addCriterion("code not like", value, "code");
return (Criteria) this;
}
public Criteria andCodeIn(List<String> values) {
addCriterion("code in", values, "code");
return (Criteria) this;
}
public Criteria andCodeNotIn(List<String> values) {
addCriterion("code not in", values, "code");
return (Criteria) this;
}
public Criteria andCodeBetween(String value1, String value2) {
addCriterion("code between", value1, value2, "code");
return (Criteria) this;
}
public Criteria andCodeNotBetween(String value1, String value2) {
addCriterion("code not between", value1, value2, "code");
return (Criteria) this;
}
public Criteria andTitleIsNull() {
addCriterion("title is null");
return (Criteria) this;
}
public Criteria andTitleIsNotNull() {
addCriterion("title is not null");
return (Criteria) this;
}
public Criteria andTitleEqualTo(String value) {
addCriterion("title =", value, "title");
return (Criteria) this;
}
public Criteria andTitleNotEqualTo(String value) {
addCriterion("title <>", value, "title");
return (Criteria) this;
}
public Criteria andTitleGreaterThan(String value) {
addCriterion("title >", value, "title");
return (Criteria) this;
}
public Criteria andTitleGreaterThanOrEqualTo(String value) {
addCriterion("title >=", value, "title");
return (Criteria) this;
}
public Criteria andTitleLessThan(String value) {
addCriterion("title <", value, "title");
return (Criteria) this;
}
public Criteria andTitleLessThanOrEqualTo(String value) {
addCriterion("title <=", value, "title");
return (Criteria) this;
}
public Criteria andTitleLike(String value) {
addCriterion("title like", value, "title");
return (Criteria) this;
}
public Criteria andTitleNotLike(String value) {
addCriterion("title not like", value, "title");
return (Criteria) this;
}
public Criteria andTitleIn(List<String> values) {
addCriterion("title in", values, "title");
return (Criteria) this;
}
public Criteria andTitleNotIn(List<String> values) {
addCriterion("title not in", values, "title");
return (Criteria) this;
}
public Criteria andTitleBetween(String value1, String value2) {
addCriterion("title between", value1, value2, "title");
return (Criteria) this;
}
public Criteria andTitleNotBetween(String value1, String value2) {
addCriterion("title not between", value1, value2, "title");
return (Criteria) this;
}
public Criteria andAuthorIsNull() {
addCriterion("author is null");
return (Criteria) this;
}
public Criteria andAuthorIsNotNull() {
addCriterion("author is not null");
return (Criteria) this;
}
public Criteria andAuthorEqualTo(String value) {
addCriterion("author =", value, "author");
return (Criteria) this;
}
public Criteria andAuthorNotEqualTo(String value) {
addCriterion("author <>", value, "author");
return (Criteria) this;
}
public Criteria andAuthorGreaterThan(String value) {
addCriterion("author >", value, "author");
return (Criteria) this;
}
public Criteria andAuthorGreaterThanOrEqualTo(String value) {
addCriterion("author >=", value, "author");
return (Criteria) this;
}
public Criteria andAuthorLessThan(String value) {
addCriterion("author <", value, "author");
return (Criteria) this;
}
public Criteria andAuthorLessThanOrEqualTo(String value) {
addCriterion("author <=", value, "author");
return (Criteria) this;
}
public Criteria andAuthorLike(String value) {
addCriterion("author like", value, "author");
return (Criteria) this;
}
public Criteria andAuthorNotLike(String value) {
addCriterion("author not like", value, "author");
return (Criteria) this;
}
public Criteria andAuthorIn(List<String> values) {
addCriterion("author in", values, "author");
return (Criteria) this;
}
public Criteria andAuthorNotIn(List<String> values) {
addCriterion("author not in", values, "author");
return (Criteria) this;
}
public Criteria andAuthorBetween(String value1, String value2) {
addCriterion("author between", value1, value2, "author");
return (Criteria) this;
}
public Criteria andAuthorNotBetween(String value1, String value2) {
addCriterion("author not between", value1, value2, "author");
return (Criteria) this;
}
public Criteria andAuthor_emailIsNull() {
addCriterion("author_email is null");
return (Criteria) this;
}
public Criteria andAuthor_emailIsNotNull() {
addCriterion("author_email is not null");
return (Criteria) this;
}
public Criteria andAuthor_emailEqualTo(String value) {
addCriterion("author_email =", value, "author_email");
return (Criteria) this;
}
public Criteria andAuthor_emailNotEqualTo(String value) {
addCriterion("author_email <>", value, "author_email");
return (Criteria) this;
}
public Criteria andAuthor_emailGreaterThan(String value) {
addCriterion("author_email >", value, "author_email");
return (Criteria) this;
}
public Criteria andAuthor_emailGreaterThanOrEqualTo(String value) {
addCriterion("author_email >=", value, "author_email");
return (Criteria) this;
}
public Criteria andAuthor_emailLessThan(String value) {
addCriterion("author_email <", value, "author_email");
return (Criteria) this;
}
public Criteria andAuthor_emailLessThanOrEqualTo(String value) {
addCriterion("author_email <=", value, "author_email");
return (Criteria) this;
}
public Criteria andAuthor_emailLike(String value) {
addCriterion("author_email like", value, "author_email");
return (Criteria) this;
}
public Criteria andAuthor_emailNotLike(String value) {
addCriterion("author_email not like", value, "author_email");
return (Criteria) this;
}
public Criteria andAuthor_emailIn(List<String> values) {
addCriterion("author_email in", values, "author_email");
return (Criteria) this;
}
public Criteria andAuthor_emailNotIn(List<String> values) {
addCriterion("author_email not in", values, "author_email");
return (Criteria) this;
}
public Criteria andAuthor_emailBetween(String value1, String value2) {
addCriterion("author_email between", value1, value2, "author_email");
return (Criteria) this;
}
public Criteria andAuthor_emailNotBetween(String value1, String value2) {
addCriterion("author_email not between", value1, value2, "author_email");
return (Criteria) this;
}
public Criteria andArticle_dateIsNull() {
addCriterion("article_date is null");
return (Criteria) this;
}
public Criteria andArticle_dateIsNotNull() {
addCriterion("article_date is not null");
return (Criteria) this;
}
public Criteria andArticle_dateEqualTo(Date value) {
addCriterion("article_date =", value, "article_date");
return (Criteria) this;
}
public Criteria andArticle_dateNotEqualTo(Date value) {
addCriterion("article_date <>", value, "article_date");
return (Criteria) this;
}
public Criteria andArticle_dateGreaterThan(Date value) {
addCriterion("article_date >", value, "article_date");
return (Criteria) this;
}
public Criteria andArticle_dateGreaterThanOrEqualTo(Date value) {
addCriterion("article_date >=", value, "article_date");
return (Criteria) this;
}
public Criteria andArticle_dateLessThan(Date value) {
addCriterion("article_date <", value, "article_date");
return (Criteria) this;
}
public Criteria andArticle_dateLessThanOrEqualTo(Date value) {
addCriterion("article_date <=", value, "article_date");
return (Criteria) this;
}
public Criteria andArticle_dateIn(List<Date> values) {
addCriterion("article_date in", values, "article_date");
return (Criteria) this;
}
public Criteria andArticle_dateNotIn(List<Date> values) {
addCriterion("article_date not in", values, "article_date");
return (Criteria) this;
}
public Criteria andArticle_dateBetween(Date value1, Date value2) {
addCriterion("article_date between", value1, value2, "article_date");
return (Criteria) this;
}
public Criteria andArticle_dateNotBetween(Date value1, Date value2) {
addCriterion("article_date not between", value1, value2, "article_date");
return (Criteria) this;
}
public Criteria andKeywordsIsNull() {
addCriterion("keywords is null");
return (Criteria) this;
}
public Criteria andKeywordsIsNotNull() {
addCriterion("keywords is not null");
return (Criteria) this;
}
public Criteria andKeywordsEqualTo(String value) {
addCriterion("keywords =", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsNotEqualTo(String value) {
addCriterion("keywords <>", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsGreaterThan(String value) {
addCriterion("keywords >", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsGreaterThanOrEqualTo(String value) {
addCriterion("keywords >=", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsLessThan(String value) {
addCriterion("keywords <", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsLessThanOrEqualTo(String value) {
addCriterion("keywords <=", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsLike(String value) {
addCriterion("keywords like", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsNotLike(String value) {
addCriterion("keywords not like", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsIn(List<String> values) {
addCriterion("keywords in", values, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsNotIn(List<String> values) {
addCriterion("keywords not in", values, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsBetween(String value1, String value2) {
addCriterion("keywords between", value1, value2, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsNotBetween(String value1, String value2) {
addCriterion("keywords not between", value1, value2, "keywords");
return (Criteria) this;
}
public Criteria andIs_openIsNull() {
addCriterion("is_open is null");
return (Criteria) this;
}
public Criteria andIs_openIsNotNull() {
addCriterion("is_open is not null");
return (Criteria) this;
}
public Criteria andIs_openEqualTo(Short value) {
addCriterion("is_open =", value, "is_open");
return (Criteria) this;
}
public Criteria andIs_openNotEqualTo(Short value) {
addCriterion("is_open <>", value, "is_open");
return (Criteria) this;
}
public Criteria andIs_openGreaterThan(Short value) {
addCriterion("is_open >", value, "is_open");
return (Criteria) this;
}
public Criteria andIs_openGreaterThanOrEqualTo(Short value) {
addCriterion("is_open >=", value, "is_open");
return (Criteria) this;
}
public Criteria andIs_openLessThan(Short value) {
addCriterion("is_open <", value, "is_open");
return (Criteria) this;
}
public Criteria andIs_openLessThanOrEqualTo(Short value) {
addCriterion("is_open <=", value, "is_open");
return (Criteria) this;
}
public Criteria andIs_openIn(List<Short> values) {
addCriterion("is_open in", values, "is_open");
return (Criteria) this;
}
public Criteria andIs_openNotIn(List<Short> values) {
addCriterion("is_open not in", values, "is_open");
return (Criteria) this;
}
public Criteria andIs_openBetween(Short value1, Short value2) {
addCriterion("is_open between", value1, value2, "is_open");
return (Criteria) this;
}
public Criteria andIs_openNotBetween(Short value1, Short value2) {
addCriterion("is_open not between", value1, value2, "is_open");
return (Criteria) this;
}
public Criteria andCreate_dateIsNull() {
addCriterion("create_date is null");
return (Criteria) this;
}
public Criteria andCreate_dateIsNotNull() {
addCriterion("create_date is not null");
return (Criteria) this;
}
public Criteria andCreate_dateEqualTo(Date value) {
addCriterion("create_date =", value, "create_date");
return (Criteria) this;
}
public Criteria andCreate_dateNotEqualTo(Date value) {
addCriterion("create_date <>", value, "create_date");
return (Criteria) this;
}
public Criteria andCreate_dateGreaterThan(Date value) {
addCriterion("create_date >", value, "create_date");
return (Criteria) this;
}
public Criteria andCreate_dateGreaterThanOrEqualTo(Date value) {
addCriterion("create_date >=", value, "create_date");
return (Criteria) this;
}
public Criteria andCreate_dateLessThan(Date value) {
addCriterion("create_date <", value, "create_date");
return (Criteria) this;
}
public Criteria andCreate_dateLessThanOrEqualTo(Date value) {
addCriterion("create_date <=", value, "create_date");
return (Criteria) this;
}
public Criteria andCreate_dateIn(List<Date> values) {
addCriterion("create_date in", values, "create_date");
return (Criteria) this;
}
public Criteria andCreate_dateNotIn(List<Date> values) {
addCriterion("create_date not in", values, "create_date");
return (Criteria) this;
}
public Criteria andCreate_dateBetween(Date value1, Date value2) {
addCriterion("create_date between", value1, value2, "create_date");
return (Criteria) this;
}
public Criteria andCreate_dateNotBetween(Date value1, Date value2) {
addCriterion("create_date not between", value1, value2, "create_date");
return (Criteria) this;
}
public Criteria andFile_urlIsNull() {
addCriterion("file_url is null");
return (Criteria) this;
}
public Criteria andFile_urlIsNotNull() {
addCriterion("file_url is not null");
return (Criteria) this;
}
public Criteria andFile_urlEqualTo(String value) {
addCriterion("file_url =", value, "file_url");
return (Criteria) this;
}
public Criteria andFile_urlNotEqualTo(String value) {
addCriterion("file_url <>", value, "file_url");
return (Criteria) this;
}
public Criteria andFile_urlGreaterThan(String value) {
addCriterion("file_url >", value, "file_url");
return (Criteria) this;
}
public Criteria andFile_urlGreaterThanOrEqualTo(String value) {
addCriterion("file_url >=", value, "file_url");
return (Criteria) this;
}
public Criteria andFile_urlLessThan(String value) {
addCriterion("file_url <", value, "file_url");
return (Criteria) this;
}
public Criteria andFile_urlLessThanOrEqualTo(String value) {
addCriterion("file_url <=", value, "file_url");
return (Criteria) this;
}
public Criteria andFile_urlLike(String value) {
addCriterion("file_url like", value, "file_url");
return (Criteria) this;
}
public Criteria andFile_urlNotLike(String value) {
addCriterion("file_url not like", value, "file_url");
return (Criteria) this;
}
public Criteria andFile_urlIn(List<String> values) {
addCriterion("file_url in", values, "file_url");
return (Criteria) this;
}
public Criteria andFile_urlNotIn(List<String> values) {
addCriterion("file_url not in", values, "file_url");
return (Criteria) this;
}
public Criteria andFile_urlBetween(String value1, String value2) {
addCriterion("file_url between", value1, value2, "file_url");
return (Criteria) this;
}
public Criteria andFile_urlNotBetween(String value1, String value2) {
addCriterion("file_url not between", value1, value2, "file_url");
return (Criteria) this;
}
public Criteria andOpen_typeIsNull() {
addCriterion("open_type is null");
return (Criteria) this;
}
public Criteria andOpen_typeIsNotNull() {
addCriterion("open_type is not null");
return (Criteria) this;
}
public Criteria andOpen_typeEqualTo(Short value) {
addCriterion("open_type =", value, "open_type");
return (Criteria) this;
}
public Criteria andOpen_typeNotEqualTo(Short value) {
addCriterion("open_type <>", value, "open_type");
return (Criteria) this;
}
public Criteria andOpen_typeGreaterThan(Short value) {
addCriterion("open_type >", value, "open_type");
return (Criteria) this;
}
public Criteria andOpen_typeGreaterThanOrEqualTo(Short value) {
addCriterion("open_type >=", value, "open_type");
return (Criteria) this;
}
public Criteria andOpen_typeLessThan(Short value) {
addCriterion("open_type <", value, "open_type");
return (Criteria) this;
}
public Criteria andOpen_typeLessThanOrEqualTo(Short value) {
addCriterion("open_type <=", value, "open_type");
return (Criteria) this;
}
public Criteria andOpen_typeIn(List<Short> values) {
addCriterion("open_type in", values, "open_type");
return (Criteria) this;
}
public Criteria andOpen_typeNotIn(List<Short> values) {
addCriterion("open_type not in", values, "open_type");
return (Criteria) this;
}
public Criteria andOpen_typeBetween(Short value1, Short value2) {
addCriterion("open_type between", value1, value2, "open_type");
return (Criteria) this;
}
public Criteria andOpen_typeNotBetween(Short value1, Short value2) {
addCriterion("open_type not between", value1, value2, "open_type");
return (Criteria) this;
}
public Criteria andLinkIsNull() {
addCriterion("link is null");
return (Criteria) this;
}
public Criteria andLinkIsNotNull() {
addCriterion("link is not null");
return (Criteria) this;
}
public Criteria andLinkEqualTo(String value) {
addCriterion("link =", value, "link");
return (Criteria) this;
}
public Criteria andLinkNotEqualTo(String value) {
addCriterion("link <>", value, "link");
return (Criteria) this;
}
public Criteria andLinkGreaterThan(String value) {
addCriterion("link >", value, "link");
return (Criteria) this;
}
public Criteria andLinkGreaterThanOrEqualTo(String value) {
addCriterion("link >=", value, "link");
return (Criteria) this;
}
public Criteria andLinkLessThan(String value) {
addCriterion("link <", value, "link");
return (Criteria) this;
}
public Criteria andLinkLessThanOrEqualTo(String value) {
addCriterion("link <=", value, "link");
return (Criteria) this;
}
public Criteria andLinkLike(String value) {
addCriterion("link like", value, "link");
return (Criteria) this;
}
public Criteria andLinkNotLike(String value) {
addCriterion("link not like", value, "link");
return (Criteria) this;
}
public Criteria andLinkIn(List<String> values) {
addCriterion("link in", values, "link");
return (Criteria) this;
}
public Criteria andLinkNotIn(List<String> values) {
addCriterion("link not in", values, "link");
return (Criteria) this;
}
public Criteria andLinkBetween(String value1, String value2) {
addCriterion("link between", value1, value2, "link");
return (Criteria) this;
}
public Criteria andLinkNotBetween(String value1, String value2) {
addCriterion("link not between", value1, value2, "link");
return (Criteria) this;
}
public Criteria andDescriptionIsNull() {
addCriterion("description is null");
return (Criteria) this;
}
public Criteria andDescriptionIsNotNull() {
addCriterion("description is not null");
return (Criteria) this;
}
public Criteria andDescriptionEqualTo(String value) {
addCriterion("description =", value, "description");
return (Criteria) this;
}
public Criteria andDescriptionNotEqualTo(String value) {
addCriterion("description <>", value, "description");
return (Criteria) this;
}
public Criteria andDescriptionGreaterThan(String value) {
addCriterion("description >", value, "description");
return (Criteria) this;
}
public Criteria andDescriptionGreaterThanOrEqualTo(String value) {
addCriterion("description >=", value, "description");
return (Criteria) this;
}
public Criteria andDescriptionLessThan(String value) {
addCriterion("description <", value, "description");
return (Criteria) this;
}
public Criteria andDescriptionLessThanOrEqualTo(String value) {
addCriterion("description <=", value, "description");
return (Criteria) this;
}
public Criteria andDescriptionLike(String value) {
addCriterion("description like", value, "description");
return (Criteria) this;
}
public Criteria andDescriptionNotLike(String value) {
addCriterion("description not like", value, "description");
return (Criteria) this;
}
public Criteria andDescriptionIn(List<String> values) {
addCriterion("description in", values, "description");
return (Criteria) this;
}
public Criteria andDescriptionNotIn(List<String> values) {
addCriterion("description not in", values, "description");
return (Criteria) this;
}
public Criteria andDescriptionBetween(String value1, String value2) {
addCriterion("description between", value1, value2, "description");
return (Criteria) this;
}
public Criteria andDescriptionNotBetween(String value1, String value2) {
addCriterion("description not between", value1, value2, "description");
return (Criteria) this;
}
public Criteria andStore_idIsNull() {
addCriterion("store_id is null");
return (Criteria) this;
}
public Criteria andStore_idIsNotNull() {
addCriterion("store_id is not null");
return (Criteria) this;
}
public Criteria andStore_idEqualTo(Integer value) {
addCriterion("store_id =", value, "store_id");
return (Criteria) this;
}
public Criteria andStore_idNotEqualTo(Integer value) {
addCriterion("store_id <>", value, "store_id");
return (Criteria) this;
}
public Criteria andStore_idGreaterThan(Integer value) {
addCriterion("store_id >", value, "store_id");
return (Criteria) this;
}
public Criteria andStore_idGreaterThanOrEqualTo(Integer value) {
addCriterion("store_id >=", value, "store_id");
return (Criteria) this;
}
public Criteria andStore_idLessThan(Integer value) {
addCriterion("store_id <", value, "store_id");
return (Criteria) this;
}
public Criteria andStore_idLessThanOrEqualTo(Integer value) {
addCriterion("store_id <=", value, "store_id");
return (Criteria) this;
}
public Criteria andStore_idIn(List<Integer> values) {
addCriterion("store_id in", values, "store_id");
return (Criteria) this;
}
public Criteria andStore_idNotIn(List<Integer> values) {
addCriterion("store_id not in", values, "store_id");
return (Criteria) this;
}
public Criteria andStore_idBetween(Integer value1, Integer value2) {
addCriterion("store_id between", value1, value2, "store_id");
return (Criteria) this;
}
public Criteria andStore_idNotBetween(Integer value1, Integer value2) {
addCriterion("store_id not between", value1, value2, "store_id");
return (Criteria) this;
}
public Criteria andSortIsNull() {
addCriterion("sort is null");
return (Criteria) this;
}
public Criteria andSortIsNotNull() {
addCriterion("sort is not null");
return (Criteria) this;
}
public Criteria andSortEqualTo(Short value) {
addCriterion("sort =", value, "sort");
return (Criteria) this;
}
public Criteria andSortNotEqualTo(Short value) {
addCriterion("sort <>", value, "sort");
return (Criteria) this;
}
public Criteria andSortGreaterThan(Short value) {
addCriterion("sort >", value, "sort");
return (Criteria) this;
}
public Criteria andSortGreaterThanOrEqualTo(Short value) {
addCriterion("sort >=", value, "sort");
return (Criteria) this;
}
public Criteria andSortLessThan(Short value) {
addCriterion("sort <", value, "sort");
return (Criteria) this;
}
public Criteria andSortLessThanOrEqualTo(Short value) {
addCriterion("sort <=", value, "sort");
return (Criteria) this;
}
public Criteria andSortIn(List<Short> values) {
addCriterion("sort in", values, "sort");
return (Criteria) this;
}
public Criteria andSortNotIn(List<Short> values) {
addCriterion("sort not in", values, "sort");
return (Criteria) this;
}
public Criteria andSortBetween(Short value1, Short value2) {
addCriterion("sort between", value1, value2, "sort");
return (Criteria) this;
}
public Criteria andSortNotBetween(Short value1, Short value2) {
addCriterion("sort not between", value1, value2, "sort");
return (Criteria) this;
}
public Criteria andArticle_imagesIsNull() {
addCriterion("article_images is null");
return (Criteria) this;
}
public Criteria andArticle_imagesIsNotNull() {
addCriterion("article_images is not null");
return (Criteria) this;
}
public Criteria andArticle_imagesEqualTo(String value) {
addCriterion("article_images =", value, "article_images");
return (Criteria) this;
}
public Criteria andArticle_imagesNotEqualTo(String value) {
addCriterion("article_images <>", value, "article_images");
return (Criteria) this;
}
public Criteria andArticle_imagesGreaterThan(String value) {
addCriterion("article_images >", value, "article_images");
return (Criteria) this;
}
public Criteria andArticle_imagesGreaterThanOrEqualTo(String value) {
addCriterion("article_images >=", value, "article_images");
return (Criteria) this;
}
public Criteria andArticle_imagesLessThan(String value) {
addCriterion("article_images <", value, "article_images");
return (Criteria) this;
}
public Criteria andArticle_imagesLessThanOrEqualTo(String value) {
addCriterion("article_images <=", value, "article_images");
return (Criteria) this;
}
public Criteria andArticle_imagesLike(String value) {
addCriterion("article_images like", value, "article_images");
return (Criteria) this;
}
public Criteria andArticle_imagesNotLike(String value) {
addCriterion("article_images not like", value, "article_images");
return (Criteria) this;
}
public Criteria andArticle_imagesIn(List<String> values) {
addCriterion("article_images in", values, "article_images");
return (Criteria) this;
}
public Criteria andArticle_imagesNotIn(List<String> values) {
addCriterion("article_images not in", values, "article_images");
return (Criteria) this;
}
public Criteria andArticle_imagesBetween(String value1, String value2) {
addCriterion("article_images between", value1, value2, "article_images");
return (Criteria) this;
}
public Criteria andArticle_imagesNotBetween(String value1, String value2) {
addCriterion("article_images not between", value1, value2, "article_images");
return (Criteria) this;
}
public Criteria andVideo_urlIsNull() {
addCriterion("video_url is null");
return (Criteria) this;
}
public Criteria andVideo_urlIsNotNull() {
addCriterion("video_url is not null");
return (Criteria) this;
}
public Criteria andVideo_urlEqualTo(String value) {
addCriterion("video_url =", value, "video_url");
return (Criteria) this;
}
public Criteria andVideo_urlNotEqualTo(String value) {
addCriterion("video_url <>", value, "video_url");
return (Criteria) this;
}
public Criteria andVideo_urlGreaterThan(String value) {
addCriterion("video_url >", value, "video_url");
return (Criteria) this;
}
public Criteria andVideo_urlGreaterThanOrEqualTo(String value) {
addCriterion("video_url >=", value, "video_url");
return (Criteria) this;
}
public Criteria andVideo_urlLessThan(String value) {
addCriterion("video_url <", value, "video_url");
return (Criteria) this;
}
public Criteria andVideo_urlLessThanOrEqualTo(String value) {
addCriterion("video_url <=", value, "video_url");
return (Criteria) this;
}
public Criteria andVideo_urlLike(String value) {
addCriterion("video_url like", value, "video_url");
return (Criteria) this;
}
public Criteria andVideo_urlNotLike(String value) {
addCriterion("video_url not like", value, "video_url");
return (Criteria) this;
}
public Criteria andVideo_urlIn(List<String> values) {
addCriterion("video_url in", values, "video_url");
return (Criteria) this;
}
public Criteria andVideo_urlNotIn(List<String> values) {
addCriterion("video_url not in", values, "video_url");
return (Criteria) this;
}
public Criteria andVideo_urlBetween(String value1, String value2) {
addCriterion("video_url between", value1, value2, "video_url");
return (Criteria) this;
}
public Criteria andVideo_urlNotBetween(String value1, String value2) {
addCriterion("video_url not between", value1, value2, "video_url");
return (Criteria) this;
}
public Criteria andArticle_type_codeIsNull() {
addCriterion("article_type_code is null");
return (Criteria) this;
}
public Criteria andArticle_type_codeIsNotNull() {
addCriterion("article_type_code is not null");
return (Criteria) this;
}
public Criteria andArticle_type_codeEqualTo(String value) {
addCriterion("article_type_code =", value, "article_type_code");
return (Criteria) this;
}
public Criteria andArticle_type_codeNotEqualTo(String value) {
addCriterion("article_type_code <>", value, "article_type_code");
return (Criteria) this;
}
public Criteria andArticle_type_codeGreaterThan(String value) {
addCriterion("article_type_code >", value, "article_type_code");
return (Criteria) this;
}
public Criteria andArticle_type_codeGreaterThanOrEqualTo(String value) {
addCriterion("article_type_code >=", value, "article_type_code");
return (Criteria) this;
}
public Criteria andArticle_type_codeLessThan(String value) {
addCriterion("article_type_code <", value, "article_type_code");
return (Criteria) this;
}
public Criteria andArticle_type_codeLessThanOrEqualTo(String value) {
addCriterion("article_type_code <=", value, "article_type_code");
return (Criteria) this;
}
public Criteria andArticle_type_codeLike(String value) {
addCriterion("article_type_code like", value, "article_type_code");
return (Criteria) this;
}
public Criteria andArticle_type_codeNotLike(String value) {
addCriterion("article_type_code not like", value, "article_type_code");
return (Criteria) this;
}
public Criteria andArticle_type_codeIn(List<String> values) {
addCriterion("article_type_code in", values, "article_type_code");
return (Criteria) this;
}
public Criteria andArticle_type_codeNotIn(List<String> values) {
addCriterion("article_type_code not in", values, "article_type_code");
return (Criteria) this;
}
public Criteria andArticle_type_codeBetween(String value1, String value2) {
addCriterion("article_type_code between", value1, value2, "article_type_code");
return (Criteria) this;
}
public Criteria andArticle_type_codeNotBetween(String value1, String value2) {
addCriterion("article_type_code not between", value1, value2, "article_type_code");
return (Criteria) this;
}
public Criteria andUser_idIsNull() {
addCriterion("user_id is null");
return (Criteria) this;
}
public Criteria andUser_idIsNotNull() {
addCriterion("user_id is not null");
return (Criteria) this;
}
public Criteria andUser_idEqualTo(Long value) {
addCriterion("user_id =", value, "user_id");
return (Criteria) this;
}
public Criteria andUser_idNotEqualTo(Long value) {
addCriterion("user_id <>", value, "user_id");
return (Criteria) this;
}
public Criteria andUser_idGreaterThan(Long value) {
addCriterion("user_id >", value, "user_id");
return (Criteria) this;
}
public Criteria andUser_idGreaterThanOrEqualTo(Long value) {
addCriterion("user_id >=", value, "user_id");
return (Criteria) this;
}
public Criteria andUser_idLessThan(Long value) {
addCriterion("user_id <", value, "user_id");
return (Criteria) this;
}
public Criteria andUser_idLessThanOrEqualTo(Long value) {
addCriterion("user_id <=", value, "user_id");
return (Criteria) this;
}
public Criteria andUser_idIn(List<Long> values) {
addCriterion("user_id in", values, "user_id");
return (Criteria) this;
}
public Criteria andUser_idNotIn(List<Long> values) {
addCriterion("user_id not in", values, "user_id");
return (Criteria) this;
}
public Criteria andUser_idBetween(Long value1, Long value2) {
addCriterion("user_id between", value1, value2, "user_id");
return (Criteria) this;
}
public Criteria andUser_idNotBetween(Long value1, Long value2) {
addCriterion("user_id not between", value1, value2, "user_id");
return (Criteria) this;
}
public Criteria andUpdate_dateIsNull() {
addCriterion("update_date is null");
return (Criteria) this;
}
public Criteria andUpdate_dateIsNotNull() {
addCriterion("update_date is not null");
return (Criteria) this;
}
public Criteria andUpdate_dateEqualTo(Date value) {
addCriterion("update_date =", value, "update_date");
return (Criteria) this;
}
public Criteria andUpdate_dateNotEqualTo(Date value) {
addCriterion("update_date <>", value, "update_date");
return (Criteria) this;
}
public Criteria andUpdate_dateGreaterThan(Date value) {
addCriterion("update_date >", value, "update_date");
return (Criteria) this;
}
public Criteria andUpdate_dateGreaterThanOrEqualTo(Date value) {
addCriterion("update_date >=", value, "update_date");
return (Criteria) this;
}
public Criteria andUpdate_dateLessThan(Date value) {
addCriterion("update_date <", value, "update_date");
return (Criteria) this;
}
public Criteria andUpdate_dateLessThanOrEqualTo(Date value) {
addCriterion("update_date <=", value, "update_date");
return (Criteria) this;
}
public Criteria andUpdate_dateIn(List<Date> values) {
addCriterion("update_date in", values, "update_date");
return (Criteria) this;
}
public Criteria andUpdate_dateNotIn(List<Date> values) {
addCriterion("update_date not in", values, "update_date");
return (Criteria) this;
}
public Criteria andUpdate_dateBetween(Date value1, Date value2) {
addCriterion("update_date between", value1, value2, "update_date");
return (Criteria) this;
}
public Criteria andUpdate_dateNotBetween(Date value1, Date value2) {
addCriterion("update_date not between", value1, value2, "update_date");
return (Criteria) this;
}
public Criteria andArticle_enumIsNull() {
addCriterion("article_enum is null");
return (Criteria) this;
}
public Criteria andArticle_enumIsNotNull() {
addCriterion("article_enum is not null");
return (Criteria) this;
}
public Criteria andArticle_enumEqualTo(String value) {
addCriterion("article_enum =", value, "article_enum");
return (Criteria) this;
}
public Criteria andArticle_enumNotEqualTo(String value) {
addCriterion("article_enum <>", value, "article_enum");
return (Criteria) this;
}
public Criteria andArticle_enumGreaterThan(String value) {
addCriterion("article_enum >", value, "article_enum");
return (Criteria) this;
}
public Criteria andArticle_enumGreaterThanOrEqualTo(String value) {
addCriterion("article_enum >=", value, "article_enum");
return (Criteria) this;
}
public Criteria andArticle_enumLessThan(String value) {
addCriterion("article_enum <", value, "article_enum");
return (Criteria) this;
}
public Criteria andArticle_enumLessThanOrEqualTo(String value) {
addCriterion("article_enum <=", value, "article_enum");
return (Criteria) this;
}
public Criteria andArticle_enumLike(String value) {
addCriterion("article_enum like", value, "article_enum");
return (Criteria) this;
}
public Criteria andArticle_enumNotLike(String value) {
addCriterion("article_enum not like", value, "article_enum");
return (Criteria) this;
}
public Criteria andArticle_enumIn(List<String> values) {
addCriterion("article_enum in", values, "article_enum");
return (Criteria) this;
}
public Criteria andArticle_enumNotIn(List<String> values) {
addCriterion("article_enum not in", values, "article_enum");
return (Criteria) this;
}
public Criteria andArticle_enumBetween(String value1, String value2) {
addCriterion("article_enum between", value1, value2, "article_enum");
return (Criteria) this;
}
public Criteria andArticle_enumNotBetween(String value1, String value2) {
addCriterion("article_enum not between", value1, value2, "article_enum");
return (Criteria) this;
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table hai_article
*
* @mbg.generated do_not_delete_during_merge Wed Dec 07 15:37:16 CST 2016
*/
public static class Criteria extends GeneratedCriteria {
protected Criteria() {
super();
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table hai_article
*
* @mbg.generated Wed Dec 07 15:37:16 CST 2016
*/
public static class Criterion {
private String condition;
private Object value;
private Object secondValue;
private boolean noValue;
private boolean singleValue;
private boolean betweenValue;
private boolean listValue;
private String typeHandler;
public String getCondition() {
return condition;
}
public Object getValue() {
return value;
}
public Object getSecondValue() {
return secondValue;
}
public boolean isNoValue() {
return noValue;
}
public boolean isSingleValue() {
return singleValue;
}
public boolean isBetweenValue() {
return betweenValue;
}
public boolean isListValue() {
return listValue;
}
public String getTypeHandler() {
return typeHandler;
}
protected Criterion(String condition) {
super();
this.condition = condition;
this.typeHandler = null;
this.noValue = true;
}
protected Criterion(String condition, Object value, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.typeHandler = typeHandler;
if (value instanceof List<?>) {
this.listValue = true;
} else {
this.singleValue = true;
}
}
protected Criterion(String condition, Object value) {
this(condition, value, null);
}
protected Criterion(String condition, Object value, Object secondValue, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.secondValue = secondValue;
this.typeHandler = typeHandler;
this.betweenValue = true;
}
protected Criterion(String condition, Object value, Object secondValue) {
this(condition, value, secondValue, null);
}
}
}<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/service/GxrcService.java
package com.ehais.hrlucene.service;
public interface GxrcService {
/**
* @描述 检索广西人才网
* @throws Exception
* @作者 lgj628
* @日期 2016年11月19日
* @返回 void
*/
public void loadGxrc() throws Exception;
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/HaiCategory.java
package com.ehais.figoarticle.model;
import java.io.Serializable;
import java.util.List;
public class HaiCategory implements Serializable {
private List<HaiCategory> children;
public List<HaiCategory> getChildren() {
return children;
}
public void setChildren(List<HaiCategory> children) {
this.children = children;
}
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.cat_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer catId;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.cat_name
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String catName;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.keywords
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String keywords;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.cat_desc
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String catDesc;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.parent_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer parentId;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.sort_order
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Short sortOrder;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.is_show
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Boolean isShow;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.category_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String categoryUrl;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.cid
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer cid;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.created
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer created;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.updated
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer updated;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.deleted
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer deleted;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.website_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private Integer websiteId;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.calculate
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String calculate;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.spider_source
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String spiderSource;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_category.brand_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private String brandId;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
private static final long serialVersionUID = 1L;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.cat_id
*
* @return the value of hai_category.cat_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getCatId() {
return catId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.cat_id
*
* @param catId the value for hai_category.cat_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setCatId(Integer catId) {
this.catId = catId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.cat_name
*
* @return the value of hai_category.cat_name
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getCatName() {
return catName;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.cat_name
*
* @param catName the value for hai_category.cat_name
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setCatName(String catName) {
this.catName = catName;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.keywords
*
* @return the value of hai_category.keywords
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getKeywords() {
return keywords;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.keywords
*
* @param keywords the value for hai_category.keywords
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setKeywords(String keywords) {
this.keywords = keywords;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.cat_desc
*
* @return the value of hai_category.cat_desc
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getCatDesc() {
return catDesc;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.cat_desc
*
* @param catDesc the value for hai_category.cat_desc
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setCatDesc(String catDesc) {
this.catDesc = catDesc;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.parent_id
*
* @return the value of hai_category.parent_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getParentId() {
return parentId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.parent_id
*
* @param parentId the value for hai_category.parent_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setParentId(Integer parentId) {
this.parentId = parentId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.sort_order
*
* @return the value of hai_category.sort_order
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Short getSortOrder() {
return sortOrder;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.sort_order
*
* @param sortOrder the value for hai_category.sort_order
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setSortOrder(Short sortOrder) {
this.sortOrder = sortOrder;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.is_show
*
* @return the value of hai_category.is_show
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Boolean getIsShow() {
return isShow;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.is_show
*
* @param isShow the value for hai_category.is_show
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setIsShow(Boolean isShow) {
this.isShow = isShow;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.category_url
*
* @return the value of hai_category.category_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getCategoryUrl() {
return categoryUrl;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.category_url
*
* @param categoryUrl the value for hai_category.category_url
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setCategoryUrl(String categoryUrl) {
this.categoryUrl = categoryUrl;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.cid
*
* @return the value of hai_category.cid
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getCid() {
return cid;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.cid
*
* @param cid the value for hai_category.cid
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setCid(Integer cid) {
this.cid = cid;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.created
*
* @return the value of hai_category.created
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getCreated() {
return created;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.created
*
* @param created the value for hai_category.created
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setCreated(Integer created) {
this.created = created;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.updated
*
* @return the value of hai_category.updated
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getUpdated() {
return updated;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.updated
*
* @param updated the value for hai_category.updated
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setUpdated(Integer updated) {
this.updated = updated;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.deleted
*
* @return the value of hai_category.deleted
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getDeleted() {
return deleted;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.deleted
*
* @param deleted the value for hai_category.deleted
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setDeleted(Integer deleted) {
this.deleted = deleted;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.website_id
*
* @return the value of hai_category.website_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Integer getWebsiteId() {
return websiteId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.website_id
*
* @param websiteId the value for hai_category.website_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setWebsiteId(Integer websiteId) {
this.websiteId = websiteId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.calculate
*
* @return the value of hai_category.calculate
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getCalculate() {
return calculate;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.calculate
*
* @param calculate the value for hai_category.calculate
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setCalculate(String calculate) {
this.calculate = calculate;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.spider_source
*
* @return the value of hai_category.spider_source
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getSpiderSource() {
return spiderSource;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.spider_source
*
* @param spiderSource the value for hai_category.spider_source
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setSpiderSource(String spiderSource) {
this.spiderSource = spiderSource;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_category.brand_id
*
* @return the value of hai_category.brand_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getBrandId() {
return brandId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_category.brand_id
*
* @param brandId the value for hai_category.brand_id
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setBrandId(String brandId) {
this.brandId = brandId;
}
}<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/mapper/ExcelMapper.java
package com.ehais.figoarticle.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
import com.ehais.figoarticle.model.Excel;
import com.ehais.figoarticle.model.ExcelExample;
public interface ExcelMapper {
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
@Select("select count(*) from excel where #{field} = #{value} ")
int unique(@Param("field") String field, @Param("value") String value);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
@Select("select count(*) from excel where #{field} = #{value} and store_id = #{store_id}")
int uniqueStore(@Param("field") String field, @Param("value") String value, @Param("store_id") Integer store_id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
int countByExample(ExcelExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
int deleteByExample(ExcelExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
int deleteByPrimaryKey(Integer id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
int insert(Excel record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
int insertSelective(Excel record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
List<Excel> selectByExample(ExcelExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
Excel selectByPrimaryKey(Integer id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
int updateByExampleSelective(@Param("record") Excel record, @Param("example") ExcelExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
int updateByExample(@Param("record") Excel record, @Param("example") ExcelExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
int updateByPrimaryKeySelective(Excel record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
int updateByPrimaryKey(Excel record);
}<file_sep>/eh-common/src/main/java/org/ehais/model/WxUsersExample.java
package org.ehais.model;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
public class WxUsersExample {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
protected String orderByClause;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
protected boolean distinct;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
protected List<Criteria> oredCriteria;
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public WxUsersExample() {
oredCriteria = new ArrayList<Criteria>();
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getOrderByClause() {
return orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public boolean isDistinct() {
return distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
protected void addCriterionForJDBCDate(String condition, Date value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
addCriterion(condition, new java.sql.Date(value.getTime()), property);
}
protected void addCriterionForJDBCDate(String condition, List<Date> values, String property) {
if (values == null || values.size() == 0) {
throw new RuntimeException("Value list for " + property + " cannot be null or empty");
}
List<java.sql.Date> dateList = new ArrayList<java.sql.Date>();
Iterator<Date> iter = values.iterator();
while (iter.hasNext()) {
dateList.add(new java.sql.Date(iter.next().getTime()));
}
addCriterion(condition, dateList, property);
}
protected void addCriterionForJDBCDate(String condition, Date value1, Date value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
addCriterion(condition, new java.sql.Date(value1.getTime()), new java.sql.Date(value2.getTime()), property);
}
public Criteria andUser_idIsNull() {
addCriterion("user_id is null");
return (Criteria) this;
}
public Criteria andUser_idIsNotNull() {
addCriterion("user_id is not null");
return (Criteria) this;
}
public Criteria andUser_idEqualTo(Integer value) {
addCriterion("user_id =", value, "user_id");
return (Criteria) this;
}
public Criteria andUser_idNotEqualTo(Integer value) {
addCriterion("user_id <>", value, "user_id");
return (Criteria) this;
}
public Criteria andUser_idGreaterThan(Integer value) {
addCriterion("user_id >", value, "user_id");
return (Criteria) this;
}
public Criteria andUser_idGreaterThanOrEqualTo(Integer value) {
addCriterion("user_id >=", value, "user_id");
return (Criteria) this;
}
public Criteria andUser_idLessThan(Integer value) {
addCriterion("user_id <", value, "user_id");
return (Criteria) this;
}
public Criteria andUser_idLessThanOrEqualTo(Integer value) {
addCriterion("user_id <=", value, "user_id");
return (Criteria) this;
}
public Criteria andUser_idIn(List<Integer> values) {
addCriterion("user_id in", values, "user_id");
return (Criteria) this;
}
public Criteria andUser_idNotIn(List<Integer> values) {
addCriterion("user_id not in", values, "user_id");
return (Criteria) this;
}
public Criteria andUser_idBetween(Integer value1, Integer value2) {
addCriterion("user_id between", value1, value2, "user_id");
return (Criteria) this;
}
public Criteria andUser_idNotBetween(Integer value1, Integer value2) {
addCriterion("user_id not between", value1, value2, "user_id");
return (Criteria) this;
}
public Criteria andEmailIsNull() {
addCriterion("email is null");
return (Criteria) this;
}
public Criteria andEmailIsNotNull() {
addCriterion("email is not null");
return (Criteria) this;
}
public Criteria andEmailEqualTo(String value) {
addCriterion("email =", value, "email");
return (Criteria) this;
}
public Criteria andEmailNotEqualTo(String value) {
addCriterion("email <>", value, "email");
return (Criteria) this;
}
public Criteria andEmailGreaterThan(String value) {
addCriterion("email >", value, "email");
return (Criteria) this;
}
public Criteria andEmailGreaterThanOrEqualTo(String value) {
addCriterion("email >=", value, "email");
return (Criteria) this;
}
public Criteria andEmailLessThan(String value) {
addCriterion("email <", value, "email");
return (Criteria) this;
}
public Criteria andEmailLessThanOrEqualTo(String value) {
addCriterion("email <=", value, "email");
return (Criteria) this;
}
public Criteria andEmailLike(String value) {
addCriterion("email like", value, "email");
return (Criteria) this;
}
public Criteria andEmailNotLike(String value) {
addCriterion("email not like", value, "email");
return (Criteria) this;
}
public Criteria andEmailIn(List<String> values) {
addCriterion("email in", values, "email");
return (Criteria) this;
}
public Criteria andEmailNotIn(List<String> values) {
addCriterion("email not in", values, "email");
return (Criteria) this;
}
public Criteria andEmailBetween(String value1, String value2) {
addCriterion("email between", value1, value2, "email");
return (Criteria) this;
}
public Criteria andEmailNotBetween(String value1, String value2) {
addCriterion("email not between", value1, value2, "email");
return (Criteria) this;
}
public Criteria andUser_nameIsNull() {
addCriterion("user_name is null");
return (Criteria) this;
}
public Criteria andUser_nameIsNotNull() {
addCriterion("user_name is not null");
return (Criteria) this;
}
public Criteria andUser_nameEqualTo(String value) {
addCriterion("user_name =", value, "user_name");
return (Criteria) this;
}
public Criteria andUser_nameNotEqualTo(String value) {
addCriterion("user_name <>", value, "user_name");
return (Criteria) this;
}
public Criteria andUser_nameGreaterThan(String value) {
addCriterion("user_name >", value, "user_name");
return (Criteria) this;
}
public Criteria andUser_nameGreaterThanOrEqualTo(String value) {
addCriterion("user_name >=", value, "user_name");
return (Criteria) this;
}
public Criteria andUser_nameLessThan(String value) {
addCriterion("user_name <", value, "user_name");
return (Criteria) this;
}
public Criteria andUser_nameLessThanOrEqualTo(String value) {
addCriterion("user_name <=", value, "user_name");
return (Criteria) this;
}
public Criteria andUser_nameLike(String value) {
addCriterion("user_name like", value, "user_name");
return (Criteria) this;
}
public Criteria andUser_nameNotLike(String value) {
addCriterion("user_name not like", value, "user_name");
return (Criteria) this;
}
public Criteria andUser_nameIn(List<String> values) {
addCriterion("user_name in", values, "user_name");
return (Criteria) this;
}
public Criteria andUser_nameNotIn(List<String> values) {
addCriterion("user_name not in", values, "user_name");
return (Criteria) this;
}
public Criteria andUser_nameBetween(String value1, String value2) {
addCriterion("user_name between", value1, value2, "user_name");
return (Criteria) this;
}
public Criteria andUser_nameNotBetween(String value1, String value2) {
addCriterion("user_name not between", value1, value2, "user_name");
return (Criteria) this;
}
public Criteria andUser_noIsNull() {
addCriterion("user_no is null");
return (Criteria) this;
}
public Criteria andUser_noIsNotNull() {
addCriterion("user_no is not null");
return (Criteria) this;
}
public Criteria andUser_noEqualTo(String value) {
addCriterion("user_no =", value, "user_no");
return (Criteria) this;
}
public Criteria andUser_noNotEqualTo(String value) {
addCriterion("user_no <>", value, "user_no");
return (Criteria) this;
}
public Criteria andUser_noGreaterThan(String value) {
addCriterion("user_no >", value, "user_no");
return (Criteria) this;
}
public Criteria andUser_noGreaterThanOrEqualTo(String value) {
addCriterion("user_no >=", value, "user_no");
return (Criteria) this;
}
public Criteria andUser_noLessThan(String value) {
addCriterion("user_no <", value, "user_no");
return (Criteria) this;
}
public Criteria andUser_noLessThanOrEqualTo(String value) {
addCriterion("user_no <=", value, "user_no");
return (Criteria) this;
}
public Criteria andUser_noLike(String value) {
addCriterion("user_no like", value, "user_no");
return (Criteria) this;
}
public Criteria andUser_noNotLike(String value) {
addCriterion("user_no not like", value, "user_no");
return (Criteria) this;
}
public Criteria andUser_noIn(List<String> values) {
addCriterion("user_no in", values, "user_no");
return (Criteria) this;
}
public Criteria andUser_noNotIn(List<String> values) {
addCriterion("user_no not in", values, "user_no");
return (Criteria) this;
}
public Criteria andUser_noBetween(String value1, String value2) {
addCriterion("user_no between", value1, value2, "user_no");
return (Criteria) this;
}
public Criteria andUser_noNotBetween(String value1, String value2) {
addCriterion("user_no not between", value1, value2, "user_no");
return (Criteria) this;
}
public Criteria andPasswordIsNull() {
addCriterion("password is null");
return (Criteria) this;
}
public Criteria andPasswordIsNotNull() {
addCriterion("password is not null");
return (Criteria) this;
}
public Criteria andPasswordEqualTo(String value) {
addCriterion("password =", value, "password");
return (Criteria) this;
}
public Criteria andPasswordNotEqualTo(String value) {
addCriterion("password <>", value, "password");
return (Criteria) this;
}
public Criteria andPasswordGreaterThan(String value) {
addCriterion("password >", value, "password");
return (Criteria) this;
}
public Criteria andPasswordGreaterThanOrEqualTo(String value) {
addCriterion("password >=", value, "password");
return (Criteria) this;
}
public Criteria andPasswordLessThan(String value) {
addCriterion("password <", value, "<PASSWORD>");
return (Criteria) this;
}
public Criteria andPasswordLessThanOrEqualTo(String value) {
addCriterion("password <=", value, "<PASSWORD>");
return (Criteria) this;
}
public Criteria andPasswordLike(String value) {
addCriterion("password like", value, "password");
return (Criteria) this;
}
public Criteria andPasswordNotLike(String value) {
addCriterion("password not like", value, "password");
return (Criteria) this;
}
public Criteria andPasswordIn(List<String> values) {
addCriterion("password in", values, "password");
return (Criteria) this;
}
public Criteria andPasswordNotIn(List<String> values) {
addCriterion("password not in", values, "password");
return (Criteria) this;
}
public Criteria andPasswordBetween(String value1, String value2) {
addCriterion("password between", value1, value2, "password");
return (Criteria) this;
}
public Criteria andPasswordNotBetween(String value1, String value2) {
addCriterion("password not between", value1, value2, "password");
return (Criteria) this;
}
public Criteria andNicknameIsNull() {
addCriterion("nickname is null");
return (Criteria) this;
}
public Criteria andNicknameIsNotNull() {
addCriterion("nickname is not null");
return (Criteria) this;
}
public Criteria andNicknameEqualTo(String value) {
addCriterion("nickname =", value, "nickname");
return (Criteria) this;
}
public Criteria andNicknameNotEqualTo(String value) {
addCriterion("nickname <>", value, "nickname");
return (Criteria) this;
}
public Criteria andNicknameGreaterThan(String value) {
addCriterion("nickname >", value, "nickname");
return (Criteria) this;
}
public Criteria andNicknameGreaterThanOrEqualTo(String value) {
addCriterion("nickname >=", value, "nickname");
return (Criteria) this;
}
public Criteria andNicknameLessThan(String value) {
addCriterion("nickname <", value, "nickname");
return (Criteria) this;
}
public Criteria andNicknameLessThanOrEqualTo(String value) {
addCriterion("nickname <=", value, "nickname");
return (Criteria) this;
}
public Criteria andNicknameLike(String value) {
addCriterion("nickname like", value, "nickname");
return (Criteria) this;
}
public Criteria andNicknameNotLike(String value) {
addCriterion("nickname not like", value, "nickname");
return (Criteria) this;
}
public Criteria andNicknameIn(List<String> values) {
addCriterion("nickname in", values, "nickname");
return (Criteria) this;
}
public Criteria andNicknameNotIn(List<String> values) {
addCriterion("nickname not in", values, "nickname");
return (Criteria) this;
}
public Criteria andNicknameBetween(String value1, String value2) {
addCriterion("nickname between", value1, value2, "nickname");
return (Criteria) this;
}
public Criteria andNicknameNotBetween(String value1, String value2) {
addCriterion("nickname not between", value1, value2, "nickname");
return (Criteria) this;
}
public Criteria andRealnameIsNull() {
addCriterion("realname is null");
return (Criteria) this;
}
public Criteria andRealnameIsNotNull() {
addCriterion("realname is not null");
return (Criteria) this;
}
public Criteria andRealnameEqualTo(String value) {
addCriterion("realname =", value, "realname");
return (Criteria) this;
}
public Criteria andRealnameNotEqualTo(String value) {
addCriterion("realname <>", value, "realname");
return (Criteria) this;
}
public Criteria andRealnameGreaterThan(String value) {
addCriterion("realname >", value, "realname");
return (Criteria) this;
}
public Criteria andRealnameGreaterThanOrEqualTo(String value) {
addCriterion("realname >=", value, "realname");
return (Criteria) this;
}
public Criteria andRealnameLessThan(String value) {
addCriterion("realname <", value, "realname");
return (Criteria) this;
}
public Criteria andRealnameLessThanOrEqualTo(String value) {
addCriterion("realname <=", value, "realname");
return (Criteria) this;
}
public Criteria andRealnameLike(String value) {
addCriterion("realname like", value, "realname");
return (Criteria) this;
}
public Criteria andRealnameNotLike(String value) {
addCriterion("realname not like", value, "realname");
return (Criteria) this;
}
public Criteria andRealnameIn(List<String> values) {
addCriterion("realname in", values, "realname");
return (Criteria) this;
}
public Criteria andRealnameNotIn(List<String> values) {
addCriterion("realname not in", values, "realname");
return (Criteria) this;
}
public Criteria andRealnameBetween(String value1, String value2) {
addCriterion("realname between", value1, value2, "realname");
return (Criteria) this;
}
public Criteria andRealnameNotBetween(String value1, String value2) {
addCriterion("realname not between", value1, value2, "realname");
return (Criteria) this;
}
public Criteria andQuestionIsNull() {
addCriterion("question is null");
return (Criteria) this;
}
public Criteria andQuestionIsNotNull() {
addCriterion("question is not null");
return (Criteria) this;
}
public Criteria andQuestionEqualTo(String value) {
addCriterion("question =", value, "question");
return (Criteria) this;
}
public Criteria andQuestionNotEqualTo(String value) {
addCriterion("question <>", value, "question");
return (Criteria) this;
}
public Criteria andQuestionGreaterThan(String value) {
addCriterion("question >", value, "question");
return (Criteria) this;
}
public Criteria andQuestionGreaterThanOrEqualTo(String value) {
addCriterion("question >=", value, "question");
return (Criteria) this;
}
public Criteria andQuestionLessThan(String value) {
addCriterion("question <", value, "question");
return (Criteria) this;
}
public Criteria andQuestionLessThanOrEqualTo(String value) {
addCriterion("question <=", value, "question");
return (Criteria) this;
}
public Criteria andQuestionLike(String value) {
addCriterion("question like", value, "question");
return (Criteria) this;
}
public Criteria andQuestionNotLike(String value) {
addCriterion("question not like", value, "question");
return (Criteria) this;
}
public Criteria andQuestionIn(List<String> values) {
addCriterion("question in", values, "question");
return (Criteria) this;
}
public Criteria andQuestionNotIn(List<String> values) {
addCriterion("question not in", values, "question");
return (Criteria) this;
}
public Criteria andQuestionBetween(String value1, String value2) {
addCriterion("question between", value1, value2, "question");
return (Criteria) this;
}
public Criteria andQuestionNotBetween(String value1, String value2) {
addCriterion("question not between", value1, value2, "question");
return (Criteria) this;
}
public Criteria andAnswerIsNull() {
addCriterion("answer is null");
return (Criteria) this;
}
public Criteria andAnswerIsNotNull() {
addCriterion("answer is not null");
return (Criteria) this;
}
public Criteria andAnswerEqualTo(String value) {
addCriterion("answer =", value, "answer");
return (Criteria) this;
}
public Criteria andAnswerNotEqualTo(String value) {
addCriterion("answer <>", value, "answer");
return (Criteria) this;
}
public Criteria andAnswerGreaterThan(String value) {
addCriterion("answer >", value, "answer");
return (Criteria) this;
}
public Criteria andAnswerGreaterThanOrEqualTo(String value) {
addCriterion("answer >=", value, "answer");
return (Criteria) this;
}
public Criteria andAnswerLessThan(String value) {
addCriterion("answer <", value, "answer");
return (Criteria) this;
}
public Criteria andAnswerLessThanOrEqualTo(String value) {
addCriterion("answer <=", value, "answer");
return (Criteria) this;
}
public Criteria andAnswerLike(String value) {
addCriterion("answer like", value, "answer");
return (Criteria) this;
}
public Criteria andAnswerNotLike(String value) {
addCriterion("answer not like", value, "answer");
return (Criteria) this;
}
public Criteria andAnswerIn(List<String> values) {
addCriterion("answer in", values, "answer");
return (Criteria) this;
}
public Criteria andAnswerNotIn(List<String> values) {
addCriterion("answer not in", values, "answer");
return (Criteria) this;
}
public Criteria andAnswerBetween(String value1, String value2) {
addCriterion("answer between", value1, value2, "answer");
return (Criteria) this;
}
public Criteria andAnswerNotBetween(String value1, String value2) {
addCriterion("answer not between", value1, value2, "answer");
return (Criteria) this;
}
public Criteria andSexIsNull() {
addCriterion("sex is null");
return (Criteria) this;
}
public Criteria andSexIsNotNull() {
addCriterion("sex is not null");
return (Criteria) this;
}
public Criteria andSexEqualTo(Boolean value) {
addCriterion("sex =", value, "sex");
return (Criteria) this;
}
public Criteria andSexNotEqualTo(Boolean value) {
addCriterion("sex <>", value, "sex");
return (Criteria) this;
}
public Criteria andSexGreaterThan(Boolean value) {
addCriterion("sex >", value, "sex");
return (Criteria) this;
}
public Criteria andSexGreaterThanOrEqualTo(Boolean value) {
addCriterion("sex >=", value, "sex");
return (Criteria) this;
}
public Criteria andSexLessThan(Boolean value) {
addCriterion("sex <", value, "sex");
return (Criteria) this;
}
public Criteria andSexLessThanOrEqualTo(Boolean value) {
addCriterion("sex <=", value, "sex");
return (Criteria) this;
}
public Criteria andSexIn(List<Boolean> values) {
addCriterion("sex in", values, "sex");
return (Criteria) this;
}
public Criteria andSexNotIn(List<Boolean> values) {
addCriterion("sex not in", values, "sex");
return (Criteria) this;
}
public Criteria andSexBetween(Boolean value1, Boolean value2) {
addCriterion("sex between", value1, value2, "sex");
return (Criteria) this;
}
public Criteria andSexNotBetween(Boolean value1, Boolean value2) {
addCriterion("sex not between", value1, value2, "sex");
return (Criteria) this;
}
public Criteria andBirthdayIsNull() {
addCriterion("birthday is null");
return (Criteria) this;
}
public Criteria andBirthdayIsNotNull() {
addCriterion("birthday is not null");
return (Criteria) this;
}
public Criteria andBirthdayEqualTo(Date value) {
addCriterionForJDBCDate("birthday =", value, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayNotEqualTo(Date value) {
addCriterionForJDBCDate("birthday <>", value, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayGreaterThan(Date value) {
addCriterionForJDBCDate("birthday >", value, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayGreaterThanOrEqualTo(Date value) {
addCriterionForJDBCDate("birthday >=", value, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayLessThan(Date value) {
addCriterionForJDBCDate("birthday <", value, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayLessThanOrEqualTo(Date value) {
addCriterionForJDBCDate("birthday <=", value, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayIn(List<Date> values) {
addCriterionForJDBCDate("birthday in", values, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayNotIn(List<Date> values) {
addCriterionForJDBCDate("birthday not in", values, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayBetween(Date value1, Date value2) {
addCriterionForJDBCDate("birthday between", value1, value2, "birthday");
return (Criteria) this;
}
public Criteria andBirthdayNotBetween(Date value1, Date value2) {
addCriterionForJDBCDate("birthday not between", value1, value2, "birthday");
return (Criteria) this;
}
public Criteria andReg_timeIsNull() {
addCriterion("reg_time is null");
return (Criteria) this;
}
public Criteria andReg_timeIsNotNull() {
addCriterion("reg_time is not null");
return (Criteria) this;
}
public Criteria andReg_timeEqualTo(Date value) {
addCriterion("reg_time =", value, "reg_time");
return (Criteria) this;
}
public Criteria andReg_timeNotEqualTo(Date value) {
addCriterion("reg_time <>", value, "reg_time");
return (Criteria) this;
}
public Criteria andReg_timeGreaterThan(Date value) {
addCriterion("reg_time >", value, "reg_time");
return (Criteria) this;
}
public Criteria andReg_timeGreaterThanOrEqualTo(Date value) {
addCriterion("reg_time >=", value, "reg_time");
return (Criteria) this;
}
public Criteria andReg_timeLessThan(Date value) {
addCriterion("reg_time <", value, "reg_time");
return (Criteria) this;
}
public Criteria andReg_timeLessThanOrEqualTo(Date value) {
addCriterion("reg_time <=", value, "reg_time");
return (Criteria) this;
}
public Criteria andReg_timeIn(List<Date> values) {
addCriterion("reg_time in", values, "reg_time");
return (Criteria) this;
}
public Criteria andReg_timeNotIn(List<Date> values) {
addCriterion("reg_time not in", values, "reg_time");
return (Criteria) this;
}
public Criteria andReg_timeBetween(Date value1, Date value2) {
addCriterion("reg_time between", value1, value2, "reg_time");
return (Criteria) this;
}
public Criteria andReg_timeNotBetween(Date value1, Date value2) {
addCriterion("reg_time not between", value1, value2, "reg_time");
return (Criteria) this;
}
public Criteria andLast_loginIsNull() {
addCriterion("last_login is null");
return (Criteria) this;
}
public Criteria andLast_loginIsNotNull() {
addCriterion("last_login is not null");
return (Criteria) this;
}
public Criteria andLast_loginEqualTo(Date value) {
addCriterion("last_login =", value, "last_login");
return (Criteria) this;
}
public Criteria andLast_loginNotEqualTo(Date value) {
addCriterion("last_login <>", value, "last_login");
return (Criteria) this;
}
public Criteria andLast_loginGreaterThan(Date value) {
addCriterion("last_login >", value, "last_login");
return (Criteria) this;
}
public Criteria andLast_loginGreaterThanOrEqualTo(Date value) {
addCriterion("last_login >=", value, "last_login");
return (Criteria) this;
}
public Criteria andLast_loginLessThan(Date value) {
addCriterion("last_login <", value, "last_login");
return (Criteria) this;
}
public Criteria andLast_loginLessThanOrEqualTo(Date value) {
addCriterion("last_login <=", value, "last_login");
return (Criteria) this;
}
public Criteria andLast_loginIn(List<Date> values) {
addCriterion("last_login in", values, "last_login");
return (Criteria) this;
}
public Criteria andLast_loginNotIn(List<Date> values) {
addCriterion("last_login not in", values, "last_login");
return (Criteria) this;
}
public Criteria andLast_loginBetween(Date value1, Date value2) {
addCriterion("last_login between", value1, value2, "last_login");
return (Criteria) this;
}
public Criteria andLast_loginNotBetween(Date value1, Date value2) {
addCriterion("last_login not between", value1, value2, "last_login");
return (Criteria) this;
}
public Criteria andLast_ipIsNull() {
addCriterion("last_ip is null");
return (Criteria) this;
}
public Criteria andLast_ipIsNotNull() {
addCriterion("last_ip is not null");
return (Criteria) this;
}
public Criteria andLast_ipEqualTo(String value) {
addCriterion("last_ip =", value, "last_ip");
return (Criteria) this;
}
public Criteria andLast_ipNotEqualTo(String value) {
addCriterion("last_ip <>", value, "last_ip");
return (Criteria) this;
}
public Criteria andLast_ipGreaterThan(String value) {
addCriterion("last_ip >", value, "last_ip");
return (Criteria) this;
}
public Criteria andLast_ipGreaterThanOrEqualTo(String value) {
addCriterion("last_ip >=", value, "last_ip");
return (Criteria) this;
}
public Criteria andLast_ipLessThan(String value) {
addCriterion("last_ip <", value, "last_ip");
return (Criteria) this;
}
public Criteria andLast_ipLessThanOrEqualTo(String value) {
addCriterion("last_ip <=", value, "last_ip");
return (Criteria) this;
}
public Criteria andLast_ipLike(String value) {
addCriterion("last_ip like", value, "last_ip");
return (Criteria) this;
}
public Criteria andLast_ipNotLike(String value) {
addCriterion("last_ip not like", value, "last_ip");
return (Criteria) this;
}
public Criteria andLast_ipIn(List<String> values) {
addCriterion("last_ip in", values, "last_ip");
return (Criteria) this;
}
public Criteria andLast_ipNotIn(List<String> values) {
addCriterion("last_ip not in", values, "last_ip");
return (Criteria) this;
}
public Criteria andLast_ipBetween(String value1, String value2) {
addCriterion("last_ip between", value1, value2, "last_ip");
return (Criteria) this;
}
public Criteria andLast_ipNotBetween(String value1, String value2) {
addCriterion("last_ip not between", value1, value2, "last_ip");
return (Criteria) this;
}
public Criteria andVisit_countIsNull() {
addCriterion("visit_count is null");
return (Criteria) this;
}
public Criteria andVisit_countIsNotNull() {
addCriterion("visit_count is not null");
return (Criteria) this;
}
public Criteria andVisit_countEqualTo(Short value) {
addCriterion("visit_count =", value, "visit_count");
return (Criteria) this;
}
public Criteria andVisit_countNotEqualTo(Short value) {
addCriterion("visit_count <>", value, "visit_count");
return (Criteria) this;
}
public Criteria andVisit_countGreaterThan(Short value) {
addCriterion("visit_count >", value, "visit_count");
return (Criteria) this;
}
public Criteria andVisit_countGreaterThanOrEqualTo(Short value) {
addCriterion("visit_count >=", value, "visit_count");
return (Criteria) this;
}
public Criteria andVisit_countLessThan(Short value) {
addCriterion("visit_count <", value, "visit_count");
return (Criteria) this;
}
public Criteria andVisit_countLessThanOrEqualTo(Short value) {
addCriterion("visit_count <=", value, "visit_count");
return (Criteria) this;
}
public Criteria andVisit_countIn(List<Short> values) {
addCriterion("visit_count in", values, "visit_count");
return (Criteria) this;
}
public Criteria andVisit_countNotIn(List<Short> values) {
addCriterion("visit_count not in", values, "visit_count");
return (Criteria) this;
}
public Criteria andVisit_countBetween(Short value1, Short value2) {
addCriterion("visit_count between", value1, value2, "visit_count");
return (Criteria) this;
}
public Criteria andVisit_countNotBetween(Short value1, Short value2) {
addCriterion("visit_count not between", value1, value2, "visit_count");
return (Criteria) this;
}
public Criteria andMobileIsNull() {
addCriterion("mobile is null");
return (Criteria) this;
}
public Criteria andMobileIsNotNull() {
addCriterion("mobile is not null");
return (Criteria) this;
}
public Criteria andMobileEqualTo(String value) {
addCriterion("mobile =", value, "mobile");
return (Criteria) this;
}
public Criteria andMobileNotEqualTo(String value) {
addCriterion("mobile <>", value, "mobile");
return (Criteria) this;
}
public Criteria andMobileGreaterThan(String value) {
addCriterion("mobile >", value, "mobile");
return (Criteria) this;
}
public Criteria andMobileGreaterThanOrEqualTo(String value) {
addCriterion("mobile >=", value, "mobile");
return (Criteria) this;
}
public Criteria andMobileLessThan(String value) {
addCriterion("mobile <", value, "mobile");
return (Criteria) this;
}
public Criteria andMobileLessThanOrEqualTo(String value) {
addCriterion("mobile <=", value, "mobile");
return (Criteria) this;
}
public Criteria andMobileLike(String value) {
addCriterion("mobile like", value, "mobile");
return (Criteria) this;
}
public Criteria andMobileNotLike(String value) {
addCriterion("mobile not like", value, "mobile");
return (Criteria) this;
}
public Criteria andMobileIn(List<String> values) {
addCriterion("mobile in", values, "mobile");
return (Criteria) this;
}
public Criteria andMobileNotIn(List<String> values) {
addCriterion("mobile not in", values, "mobile");
return (Criteria) this;
}
public Criteria andMobileBetween(String value1, String value2) {
addCriterion("mobile between", value1, value2, "mobile");
return (Criteria) this;
}
public Criteria andMobileNotBetween(String value1, String value2) {
addCriterion("mobile not between", value1, value2, "mobile");
return (Criteria) this;
}
public Criteria andIs_validatedIsNull() {
addCriterion("is_validated is null");
return (Criteria) this;
}
public Criteria andIs_validatedIsNotNull() {
addCriterion("is_validated is not null");
return (Criteria) this;
}
public Criteria andIs_validatedEqualTo(Byte value) {
addCriterion("is_validated =", value, "is_validated");
return (Criteria) this;
}
public Criteria andIs_validatedNotEqualTo(Byte value) {
addCriterion("is_validated <>", value, "is_validated");
return (Criteria) this;
}
public Criteria andIs_validatedGreaterThan(Byte value) {
addCriterion("is_validated >", value, "is_validated");
return (Criteria) this;
}
public Criteria andIs_validatedGreaterThanOrEqualTo(Byte value) {
addCriterion("is_validated >=", value, "is_validated");
return (Criteria) this;
}
public Criteria andIs_validatedLessThan(Byte value) {
addCriterion("is_validated <", value, "is_validated");
return (Criteria) this;
}
public Criteria andIs_validatedLessThanOrEqualTo(Byte value) {
addCriterion("is_validated <=", value, "is_validated");
return (Criteria) this;
}
public Criteria andIs_validatedIn(List<Byte> values) {
addCriterion("is_validated in", values, "is_validated");
return (Criteria) this;
}
public Criteria andIs_validatedNotIn(List<Byte> values) {
addCriterion("is_validated not in", values, "is_validated");
return (Criteria) this;
}
public Criteria andIs_validatedBetween(Byte value1, Byte value2) {
addCriterion("is_validated between", value1, value2, "is_validated");
return (Criteria) this;
}
public Criteria andIs_validatedNotBetween(Byte value1, Byte value2) {
addCriterion("is_validated not between", value1, value2, "is_validated");
return (Criteria) this;
}
public Criteria andCredit_lineIsNull() {
addCriterion("credit_line is null");
return (Criteria) this;
}
public Criteria andCredit_lineIsNotNull() {
addCriterion("credit_line is not null");
return (Criteria) this;
}
public Criteria andCredit_lineEqualTo(BigDecimal value) {
addCriterion("credit_line =", value, "credit_line");
return (Criteria) this;
}
public Criteria andCredit_lineNotEqualTo(BigDecimal value) {
addCriterion("credit_line <>", value, "credit_line");
return (Criteria) this;
}
public Criteria andCredit_lineGreaterThan(BigDecimal value) {
addCriterion("credit_line >", value, "credit_line");
return (Criteria) this;
}
public Criteria andCredit_lineGreaterThanOrEqualTo(BigDecimal value) {
addCriterion("credit_line >=", value, "credit_line");
return (Criteria) this;
}
public Criteria andCredit_lineLessThan(BigDecimal value) {
addCriterion("credit_line <", value, "credit_line");
return (Criteria) this;
}
public Criteria andCredit_lineLessThanOrEqualTo(BigDecimal value) {
addCriterion("credit_line <=", value, "credit_line");
return (Criteria) this;
}
public Criteria andCredit_lineIn(List<BigDecimal> values) {
addCriterion("credit_line in", values, "credit_line");
return (Criteria) this;
}
public Criteria andCredit_lineNotIn(List<BigDecimal> values) {
addCriterion("credit_line not in", values, "credit_line");
return (Criteria) this;
}
public Criteria andCredit_lineBetween(BigDecimal value1, BigDecimal value2) {
addCriterion("credit_line between", value1, value2, "credit_line");
return (Criteria) this;
}
public Criteria andCredit_lineNotBetween(BigDecimal value1, BigDecimal value2) {
addCriterion("credit_line not between", value1, value2, "credit_line");
return (Criteria) this;
}
public Criteria andPasswd_questionIsNull() {
addCriterion("passwd_question is null");
return (Criteria) this;
}
public Criteria andPasswd_questionIsNotNull() {
addCriterion("passwd_question is not null");
return (Criteria) this;
}
public Criteria andPasswd_questionEqualTo(String value) {
addCriterion("passwd_question =", value, "passwd_question");
return (Criteria) this;
}
public Criteria andPasswd_questionNotEqualTo(String value) {
addCriterion("passwd_question <>", value, "passwd_question");
return (Criteria) this;
}
public Criteria andPasswd_questionGreaterThan(String value) {
addCriterion("passwd_question >", value, "passwd_question");
return (Criteria) this;
}
public Criteria andPasswd_questionGreaterThanOrEqualTo(String value) {
addCriterion("passwd_question >=", value, "passwd_question");
return (Criteria) this;
}
public Criteria andPasswd_questionLessThan(String value) {
addCriterion("passwd_question <", value, "passwd_question");
return (Criteria) this;
}
public Criteria andPasswd_questionLessThanOrEqualTo(String value) {
addCriterion("passwd_question <=", value, "passwd_question");
return (Criteria) this;
}
public Criteria andPasswd_questionLike(String value) {
addCriterion("passwd_question like", value, "passwd_question");
return (Criteria) this;
}
public Criteria andPasswd_questionNotLike(String value) {
addCriterion("passwd_question not like", value, "passwd_question");
return (Criteria) this;
}
public Criteria andPasswd_questionIn(List<String> values) {
addCriterion("passwd_question in", values, "passwd_question");
return (Criteria) this;
}
public Criteria andPasswd_questionNotIn(List<String> values) {
addCriterion("passwd_question not in", values, "passwd_question");
return (Criteria) this;
}
public Criteria andPasswd_questionBetween(String value1, String value2) {
addCriterion("passwd_question between", value1, value2, "passwd_question");
return (Criteria) this;
}
public Criteria andPasswd_questionNotBetween(String value1, String value2) {
addCriterion("passwd_question not between", value1, value2, "passwd_question");
return (Criteria) this;
}
public Criteria andPasswd_answerIsNull() {
addCriterion("passwd_answer is null");
return (Criteria) this;
}
public Criteria andPasswd_answerIsNotNull() {
addCriterion("passwd_answer is not null");
return (Criteria) this;
}
public Criteria andPasswd_answerEqualTo(String value) {
addCriterion("passwd_answer =", value, "passwd_answer");
return (Criteria) this;
}
public Criteria andPasswd_answerNotEqualTo(String value) {
addCriterion("passwd_answer <>", value, "passwd_answer");
return (Criteria) this;
}
public Criteria andPasswd_answerGreaterThan(String value) {
addCriterion("passwd_answer >", value, "passwd_answer");
return (Criteria) this;
}
public Criteria andPasswd_answerGreaterThanOrEqualTo(String value) {
addCriterion("passwd_answer >=", value, "passwd_answer");
return (Criteria) this;
}
public Criteria andPasswd_answerLessThan(String value) {
addCriterion("passwd_answer <", value, "passwd_answer");
return (Criteria) this;
}
public Criteria andPasswd_answerLessThanOrEqualTo(String value) {
addCriterion("passwd_answer <=", value, "passwd_answer");
return (Criteria) this;
}
public Criteria andPasswd_answerLike(String value) {
addCriterion("passwd_answer like", value, "passwd_answer");
return (Criteria) this;
}
public Criteria andPasswd_answerNotLike(String value) {
addCriterion("passwd_answer not like", value, "passwd_answer");
return (Criteria) this;
}
public Criteria andPasswd_answerIn(List<String> values) {
addCriterion("passwd_answer in", values, "passwd_answer");
return (Criteria) this;
}
public Criteria andPasswd_answerNotIn(List<String> values) {
addCriterion("passwd_answer not in", values, "passwd_answer");
return (Criteria) this;
}
public Criteria andPasswd_answerBetween(String value1, String value2) {
addCriterion("passwd_answer between", value1, value2, "passwd_answer");
return (Criteria) this;
}
public Criteria andPasswd_answerNotBetween(String value1, String value2) {
addCriterion("passwd_answer not between", value1, value2, "passwd_answer");
return (Criteria) this;
}
public Criteria andStore_idIsNull() {
addCriterion("store_id is null");
return (Criteria) this;
}
public Criteria andStore_idIsNotNull() {
addCriterion("store_id is not null");
return (Criteria) this;
}
public Criteria andStore_idEqualTo(Integer value) {
addCriterion("store_id =", value, "store_id");
return (Criteria) this;
}
public Criteria andStore_idNotEqualTo(Integer value) {
addCriterion("store_id <>", value, "store_id");
return (Criteria) this;
}
public Criteria andStore_idGreaterThan(Integer value) {
addCriterion("store_id >", value, "store_id");
return (Criteria) this;
}
public Criteria andStore_idGreaterThanOrEqualTo(Integer value) {
addCriterion("store_id >=", value, "store_id");
return (Criteria) this;
}
public Criteria andStore_idLessThan(Integer value) {
addCriterion("store_id <", value, "store_id");
return (Criteria) this;
}
public Criteria andStore_idLessThanOrEqualTo(Integer value) {
addCriterion("store_id <=", value, "store_id");
return (Criteria) this;
}
public Criteria andStore_idIn(List<Integer> values) {
addCriterion("store_id in", values, "store_id");
return (Criteria) this;
}
public Criteria andStore_idNotIn(List<Integer> values) {
addCriterion("store_id not in", values, "store_id");
return (Criteria) this;
}
public Criteria andStore_idBetween(Integer value1, Integer value2) {
addCriterion("store_id between", value1, value2, "store_id");
return (Criteria) this;
}
public Criteria andStore_idNotBetween(Integer value1, Integer value2) {
addCriterion("store_id not between", value1, value2, "store_id");
return (Criteria) this;
}
public Criteria andFace_imageIsNull() {
addCriterion("face_image is null");
return (Criteria) this;
}
public Criteria andFace_imageIsNotNull() {
addCriterion("face_image is not null");
return (Criteria) this;
}
public Criteria andFace_imageEqualTo(String value) {
addCriterion("face_image =", value, "face_image");
return (Criteria) this;
}
public Criteria andFace_imageNotEqualTo(String value) {
addCriterion("face_image <>", value, "face_image");
return (Criteria) this;
}
public Criteria andFace_imageGreaterThan(String value) {
addCriterion("face_image >", value, "face_image");
return (Criteria) this;
}
public Criteria andFace_imageGreaterThanOrEqualTo(String value) {
addCriterion("face_image >=", value, "face_image");
return (Criteria) this;
}
public Criteria andFace_imageLessThan(String value) {
addCriterion("face_image <", value, "face_image");
return (Criteria) this;
}
public Criteria andFace_imageLessThanOrEqualTo(String value) {
addCriterion("face_image <=", value, "face_image");
return (Criteria) this;
}
public Criteria andFace_imageLike(String value) {
addCriterion("face_image like", value, "face_image");
return (Criteria) this;
}
public Criteria andFace_imageNotLike(String value) {
addCriterion("face_image not like", value, "face_image");
return (Criteria) this;
}
public Criteria andFace_imageIn(List<String> values) {
addCriterion("face_image in", values, "face_image");
return (Criteria) this;
}
public Criteria andFace_imageNotIn(List<String> values) {
addCriterion("face_image not in", values, "face_image");
return (Criteria) this;
}
public Criteria andFace_imageBetween(String value1, String value2) {
addCriterion("face_image between", value1, value2, "face_image");
return (Criteria) this;
}
public Criteria andFace_imageNotBetween(String value1, String value2) {
addCriterion("face_image not between", value1, value2, "face_image");
return (Criteria) this;
}
public Criteria andOpenidIsNull() {
addCriterion("openid is null");
return (Criteria) this;
}
public Criteria andOpenidIsNotNull() {
addCriterion("openid is not null");
return (Criteria) this;
}
public Criteria andOpenidEqualTo(String value) {
addCriterion("openid =", value, "openid");
return (Criteria) this;
}
public Criteria andOpenidNotEqualTo(String value) {
addCriterion("openid <>", value, "openid");
return (Criteria) this;
}
public Criteria andOpenidGreaterThan(String value) {
addCriterion("openid >", value, "openid");
return (Criteria) this;
}
public Criteria andOpenidGreaterThanOrEqualTo(String value) {
addCriterion("openid >=", value, "openid");
return (Criteria) this;
}
public Criteria andOpenidLessThan(String value) {
addCriterion("openid <", value, "openid");
return (Criteria) this;
}
public Criteria andOpenidLessThanOrEqualTo(String value) {
addCriterion("openid <=", value, "openid");
return (Criteria) this;
}
public Criteria andOpenidLike(String value) {
addCriterion("openid like", value, "openid");
return (Criteria) this;
}
public Criteria andOpenidNotLike(String value) {
addCriterion("openid not like", value, "openid");
return (Criteria) this;
}
public Criteria andOpenidIn(List<String> values) {
addCriterion("openid in", values, "openid");
return (Criteria) this;
}
public Criteria andOpenidNotIn(List<String> values) {
addCriterion("openid not in", values, "openid");
return (Criteria) this;
}
public Criteria andOpenidBetween(String value1, String value2) {
addCriterion("openid between", value1, value2, "openid");
return (Criteria) this;
}
public Criteria andOpenidNotBetween(String value1, String value2) {
addCriterion("openid not between", value1, value2, "openid");
return (Criteria) this;
}
public Criteria andSubscribeIsNull() {
addCriterion("subscribe is null");
return (Criteria) this;
}
public Criteria andSubscribeIsNotNull() {
addCriterion("subscribe is not null");
return (Criteria) this;
}
public Criteria andSubscribeEqualTo(Integer value) {
addCriterion("subscribe =", value, "subscribe");
return (Criteria) this;
}
public Criteria andSubscribeNotEqualTo(Integer value) {
addCriterion("subscribe <>", value, "subscribe");
return (Criteria) this;
}
public Criteria andSubscribeGreaterThan(Integer value) {
addCriterion("subscribe >", value, "subscribe");
return (Criteria) this;
}
public Criteria andSubscribeGreaterThanOrEqualTo(Integer value) {
addCriterion("subscribe >=", value, "subscribe");
return (Criteria) this;
}
public Criteria andSubscribeLessThan(Integer value) {
addCriterion("subscribe <", value, "subscribe");
return (Criteria) this;
}
public Criteria andSubscribeLessThanOrEqualTo(Integer value) {
addCriterion("subscribe <=", value, "subscribe");
return (Criteria) this;
}
public Criteria andSubscribeIn(List<Integer> values) {
addCriterion("subscribe in", values, "subscribe");
return (Criteria) this;
}
public Criteria andSubscribeNotIn(List<Integer> values) {
addCriterion("subscribe not in", values, "subscribe");
return (Criteria) this;
}
public Criteria andSubscribeBetween(Integer value1, Integer value2) {
addCriterion("subscribe between", value1, value2, "subscribe");
return (Criteria) this;
}
public Criteria andSubscribeNotBetween(Integer value1, Integer value2) {
addCriterion("subscribe not between", value1, value2, "subscribe");
return (Criteria) this;
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table wx_users
*
* @mbg.generated do_not_delete_during_merge Tue Dec 06 02:21:19 CST 2016
*/
public static class Criteria extends GeneratedCriteria {
protected Criteria() {
super();
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table wx_users
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public static class Criterion {
private String condition;
private Object value;
private Object secondValue;
private boolean noValue;
private boolean singleValue;
private boolean betweenValue;
private boolean listValue;
private String typeHandler;
public String getCondition() {
return condition;
}
public Object getValue() {
return value;
}
public Object getSecondValue() {
return secondValue;
}
public boolean isNoValue() {
return noValue;
}
public boolean isSingleValue() {
return singleValue;
}
public boolean isBetweenValue() {
return betweenValue;
}
public boolean isListValue() {
return listValue;
}
public String getTypeHandler() {
return typeHandler;
}
protected Criterion(String condition) {
super();
this.condition = condition;
this.typeHandler = null;
this.noValue = true;
}
protected Criterion(String condition, Object value, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.typeHandler = typeHandler;
if (value instanceof List<?>) {
this.listValue = true;
} else {
this.singleValue = true;
}
}
protected Criterion(String condition, Object value) {
this(condition, value, null);
}
protected Criterion(String condition, Object value, Object secondValue, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.secondValue = secondValue;
this.typeHandler = typeHandler;
this.betweenValue = true;
}
protected Criterion(String condition, Object value, Object secondValue) {
this(condition, value, secondValue, null);
}
}
}<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/SelfridgesController.java
package com.ehais.figoarticle.controller;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.logging.LogFactory;
import org.ehais.util.Bean2Utils;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.jsoup.nodes.Document;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.model.HaiCategory;
import com.ehais.figoarticle.model.HaiCategoryExample;
import com.ehais.figoarticle.model.HaiGoodsAttr;
import com.ehais.figoarticle.model.HaiGoodsEntity;
import com.ehais.figoarticle.model.HaiGoodsGallery;
import com.ehais.figoarticle.model.HaiGoodsUrl;
import com.ehais.figoarticle.model.HaiGoodsUrlExample;
import com.ehais.figoarticle.model.HaiGoodsWithBLOBs;
import com.gargoylesoftware.htmlunit.BrowserVersion;
import com.gargoylesoftware.htmlunit.NicelyResynchronizingAjaxController;
import com.gargoylesoftware.htmlunit.WebClient;
import com.gargoylesoftware.htmlunit.html.HtmlPage;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
@Controller
@RequestMapping("/selfridges")
public class SelfridgesController extends FigoCommonController{
private static String url = "http://www.selfridges.com";
private int websiteId = 16;
private int flag = 0;
@ResponseBody
@RequestMapping("/brand")
public String brand(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
return "";
}
@ResponseBody
@RequestMapping("/category")
public String category(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
this.category(request, url);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private void category(HttpServletRequest request,String categoryUrl){
String result = "";
try {
// TODO
List<HaiCategory> list = new ArrayList<HaiCategory>();
// result = GetPostTest.sendGet(categoryUrl + "/CN/en/", null);
// Document doc = Jsoup.parse(result);
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), categoryUrl + "/CN/en/");
Document doc = Jsoup.parse(result);
Element megaMenu = doc.getElementById("megaMenu");
Elements mainCategory = megaMenu.getElementsByTag("li");
for(Element category : mainCategory) {
Element catA = category.getElementsByTag("a").first();
if(catA == null)
continue;
String href = catA.attr("href");
if(href.indexOf("http") < 0)
href = url + href;
System.out.println(href);
HaiCategory cat = new HaiCategory();
cat.setCatName(catA.text());
cat.setCategoryUrl(href);
cat.setIsShow(true);
cat.setWebsiteId(websiteId);
//子分类
List<HaiCategory> catList = new ArrayList<HaiCategory>();
if(catA.text().equals("SALE") || catA.text().equals("Inspiration")) {
list.add(cat);
continue;
}
if(catA.text().equals("Jewellery & Watches")) {
Document doc1 = Jsoup.connect(href).get();
Element dpcategory = doc1.getElementsByClass("hub__container").first();
Elements as = dpcategory.getElementsByTag("a");
for(Element a : as) {
String aHref = a.attr("href");
if(aHref.indexOf("http") < 0)
aHref = url + aHref;
HaiCategory cat2 = new HaiCategory();
cat2.setCatName(a.text());
cat2.setCategoryUrl(aHref);
cat2.setIsShow(true);
cat2.setParentId(cat.getParentId());
cat2.setWebsiteId(websiteId);
catList.add(cat2);
}
cat.setChildren(catList);
list.add(cat);
continue;
}
Document doc1 = Jsoup.connect(href).get();
Elements dpcategories = doc1.getElementsByClass("dp__categories");
for(Element dpcategory : dpcategories) {
Element dpcontainer = dpcategory.getElementsByClass("dp__container").first();
Elements as = dpcontainer.getElementsByTag("a");
System.out.println(as);
for(Element a : as) {
String aHref = a.attr("href");
if(aHref.indexOf("http") < 0)
aHref = url + aHref;
HaiCategory cat2 = new HaiCategory();
cat2.setCatName(a.text());
cat2.setCategoryUrl(aHref);
cat2.setIsShow(true);
cat2.setParentId(cat.getParentId());
cat2.setWebsiteId(websiteId);
catList.add(cat2);
}
}
cat.setChildren(catList);
list.add(cat);
}
System.out.println("==========================================");
System.out.println("==========================================");
JSONArray arr = JSONArray.fromObject(list);
System.out.println(arr.toString());
System.out.println("==========================================");
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/category";
// String api = "http://localhost:8087/api/category";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
}
@ResponseBody
@RequestMapping("/url")
public String url(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
c.andCategoryUrlLike(url+"%");
List<HaiCategory> listCategory = haiCategoryMapper.selectByExample(example);
for (HaiCategory haiCategory : listCategory) {
System.out.println(haiCategory.getCategoryUrl());
this.goodsUrl(request, haiCategory.getCategoryUrl(), haiCategory.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsUrl(HttpServletRequest request,String goodsurl,Integer catId){
System.out.println(goodsurl);
String result = "";
try{
List<String> list = new ArrayList<String>();
// TODO
//Document doc = Jsoup.connect(goodsurl).timeout(10000).get();
// final WebClient webClient = new WebClient(BrowserVersion.FIREFOX_45); LogFactory.getFactory().setAttribute("org.apache.commons.logging.Log","org.apache.commons.logging.impl.NoOpLog");
// java.util.logging.Logger.getLogger("net.sourceforge.htmlunit").setLevel(java.util.logging.Level.OFF);
// webClient.getOptions().setThrowExceptionOnScriptError(false);
// webClient.getOptions().setThrowExceptionOnFailingStatusCode(false);
// // 设置webClient的相关参数
// webClient.getOptions().setJavaScriptEnabled(true);
// webClient.getOptions().setActiveXNative(false);
// webClient.getOptions().setCssEnabled(false);
// webClient.getOptions().setThrowExceptionOnScriptError(false);
// webClient.waitForBackgroundJavaScript(600*1000);
// webClient.setAjaxController(new NicelyResynchronizingAjaxController());
//
//
// // 模拟浏览器打开一个目标网址
// final HtmlPage page = webClient.getPage(goodsurl);
// // 该方法在getPage()方法之后调用才能生效
// webClient.waitForBackgroundJavaScript(1000*3);
// webClient.setJavaScriptTimeout(0);
// result = page.asXml();
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
Document doc = Jsoup.parse(result);
if(doc == null)
return "";
Element products = doc.getElementsByClass("products").first();
if(products == null) {
Element productsWrapper = doc.getElementsByClass("products-wrapper").first();
if(productsWrapper == null)
return "";
Elements productsp = productsWrapper.getElementsByClass("product");
for(Element product : productsp) {
Element la = product.getElementsByTag("a").first();
String href = la.attr("href");
if(href.indexOf("http") < 0)
href = url + href;
System.out.println(href);
list.add(href);
}
}
else {
Element productInner = products.getElementsByClass("productsInner").first();
Elements containers = productInner.getElementsByClass("productContainer");
for(Element container : containers) {
Element la = container.getElementsByTag("a").first();
String href = la.attr("href");
if(href.indexOf("http") < 0)
href = url + href;
System.out.println(href);
list.add(href);
}
}
JSONArray arr = JSONArray.fromObject(list);
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("catId", catId.toString());
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/url";
// String api = "http://localhost:8087/api/url";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
//获取下一页
Element pageSteps = doc.getElementsByClass("pageSteps").first();
Element next = pageSteps.select(".next.item.active").first();
if(next != null) {
Element aNext = next.getElementsByTag("a").first();
if(aNext == null)
return "";
this.goodsUrl(request, aNext.attr("href"), catId);
}
/* if(flag == 0) {
System.out.println("page = 1");
flag++;
//获取下一页
Element pageSteps = doc.getElementsByClass("pageSteps").first();
Elements pageLis = pageSteps.getElementsByTag("li");
if(pageSteps == null)
return "";
Element pageSelect = pageSteps.select(".item.selected").first();
Element allPages = pageLis.get(pageLis.size() - 3);
Element alla = allPages.getElementsByTag("a").first();
int index = alla.attr("href").lastIndexOf('=');
String ah = alla.attr("href").substring(0,index + 1);
int allPage = Integer.parseInt(allPages.getElementsByTag("a").first().text());
if(pageSelect.getElementsByTag("strong").text().equals("1")) {
for(int i = 2; i < allPage; i++) {
System.out.println("page = " + i);
this.goodsUrl(request, ah + i, catId);
}
flag = 0;
}
}*/
}catch(Exception e){
e.printStackTrace();
}
return result;
}
@ResponseBody
@RequestMapping("/goodsAll")
public String goodsAll(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiGoodsUrlExample example = new HaiGoodsUrlExample();
HaiGoodsUrlExample.Criteria c = example.createCriteria();
c.andGoodsUrlLike(url+"%");
c.andFinishEqualTo(false);
List<HaiGoodsUrl> listGoodsUrl = haiGoodsUrlMapper.selectByExample(example);
for (HaiGoodsUrl haiGoodsUrl : listGoodsUrl) {
goodsModel(request,haiGoodsUrl.getGoodsUrl(),haiGoodsUrl.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
public String goodsModel(HttpServletRequest request , String goodsurl ,Integer catId){
System.out.println(goodsurl);
String result = "";
HaiGoodsEntity entity = new HaiGoodsEntity();
HaiGoodsWithBLOBs goods = new HaiGoodsWithBLOBs();
List<HaiGoodsGallery> goodsGalleryList = new ArrayList<HaiGoodsGallery>();
List<HaiGoodsAttr> goodsAttrList = new ArrayList<HaiGoodsAttr>();
HaiGoodsAttr goodsAttr = new HaiGoodsAttr();
goods.setGoodsUrl(goodsurl);
goods.setCatId(catId);
goods.setWebsiteId(websiteId);
try{
// final WebClient webClient = new WebClient(BrowserVersion.FIREFOX_45); LogFactory.getFactory().setAttribute("org.apache.commons.logging.Log","org.apache.commons.logging.impl.NoOpLog");
// java.util.logging.Logger.getLogger("net.sourceforge.htmlunit").setLevel(java.util.logging.Level.OFF);
// webClient.getOptions().setThrowExceptionOnScriptError(false);
// webClient.getOptions().setThrowExceptionOnFailingStatusCode(false);
// // 设置webClient的相关参数
// webClient.getOptions().setJavaScriptEnabled(true);
// webClient.getOptions().setActiveXNative(false);
// webClient.getOptions().setCssEnabled(false);
// webClient.getOptions().setThrowExceptionOnScriptError(false);
// webClient.waitForBackgroundJavaScript(600*1000);
// webClient.setAjaxController(new NicelyResynchronizingAjaxController());
//
//
// // 模拟浏览器打开一个目标网址
// final HtmlPage page = webClient.getPage(goodsurl);
// // 该方法在getPage()方法之后调用才能生效
// webClient.waitForBackgroundJavaScript(1000*3);
// webClient.setJavaScriptTimeout(0);
// result = page.asXml();
// Document doc = Jsoup.parse(result);
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
Document doc = Jsoup.parse(result);
if(doc == null)
return "";
Element masterContent = doc.getElementById("masterContent");
Element prodDescInnerWrap = masterContent.getElementsByClass("prodDescInnerWrap").first();;
//设置商品名字
Element name = prodDescInnerWrap.getElementsByClass("description").first();
goods.setGoodsName(name.text());
System.out.println(name.text());
//设置价格
Element prices = prodDescInnerWrap.getElementsByClass("prices").first();
Element currPrice = prices.select(".price.red").first();
Elements priceSpans = currPrice.getElementsByTag("span");
String currency = priceSpans.get(0).text();
String price = priceSpans.get(1).text();
Integer pri;
int index1 = price.indexOf(',');
if(index1 >= 0) {
int index2 = price.indexOf(',');
int thousand = Integer.parseInt(price.substring(0, index2));
int index3 = price.indexOf('.');
int hundred = Integer.parseInt(price.substring(index2 + 1, index3));
pri = thousand * 1000 + hundred;
} else {
int index3 = price.indexOf('.');
pri = Integer.parseInt(price.substring(0, index3));
}
goods.setShopPrice(pri);
goods.setCurrency(currency);
System.out.println(currency + pri);
//设置颜色
Element basic = doc.getElementsByClass("basic").first();
Elements colorLis = basic.getElementsByTag("li");
for(Element colorLi : colorLis) {
Element img = colorLi.getElementsByTag("img").first();
String color = img.attr("alt");
HaiGoodsAttr haiGoodsColor = new HaiGoodsAttr();
haiGoodsColor.setAttrType("color");
haiGoodsColor.setAttrValue(color);
haiGoodsColor.setAttrPrice(pri.toString());
goodsAttrList.add(haiGoodsColor);
}
//设置size
Element attSize = doc.select(".att2.size").first();
Element sizeBasic = attSize.getElementsByClass("basic").first();
Elements sizeLis = sizeBasic.getElementsByTag("li");
for(Element sizeLi : sizeLis) {
Element span = sizeLi.getElementsByTag("span").first();
String size = span.text();
HaiGoodsAttr haiGoodsSize = new HaiGoodsAttr();
haiGoodsSize.setAttrType("size");
haiGoodsSize.setAttrValue(size);
haiGoodsSize.setAttrPrice(pri.toString());
goodsAttrList.add(haiGoodsSize);
}
//设置图片
Elements divs = doc.getElementsByClass("pImgWrap");
for(Element div : divs) {
Element img = div.getElementsByTag("img").first();
HaiGoodsGallery gallery = new HaiGoodsGallery();
gallery.setThumbUrl(img.attr("data-rvsrc"));
gallery.setImgUrl(img.attr("src"));
gallery.setImgOriginal(img.attr("src"));
goodsGalleryList.add(gallery);
}
if(goodsGalleryList.size() > 0) {
HaiGoodsGallery gallery = goodsGalleryList.get(0);
goods.setGoodsThumb(gallery.getThumbUrl());
goods.setGoodsImg(gallery.getImgUrl());
goods.setOriginalImg(gallery.getImgOriginal());
}
entity.setGoods(goods);
entity.setGoodsAttrList(goodsAttrList);
entity.setGoodsGalleryList(goodsGalleryList);
JSONObject jsonObject = JSONObject.fromObject(entity);
System.out.println(jsonObject.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", jsonObject.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/goodsAttr";
// String api = "http://localhost:8087/api/goodsAttr";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
//单商品的地址请求
@ResponseBody
@RequestMapping("/getGoodsUrl")
public String getGoodsUrl(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "catId", required = true) Integer catId,
@RequestParam(value = "goodsurl", required = true) String goodsurl
){
try{
return goodsModel(request,goodsurl,catId);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
public static void main(String[] args) throws Exception {
String goodsurl = "https://www.net-a-porter.com/cn/zh/d/Shop/Lingerie/All?cm_sp=topnav-_-clothing-_-lingerie";
DemoController ac = new DemoController();
}
}
<file_sep>/eh-common/src/main/java/org/ehais/protocol/PermissionProtocol.java
package org.ehais.protocol;
public final class PermissionProtocol {
public static final String URL = "url";
public static final String MENU = "menu";
public static final String BUTTON = "btn";
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/Excel.java
package com.ehais.figoarticle.model;
import java.io.Serializable;
public class Excel implements Serializable {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column excel.id
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
private Integer id;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column excel.url
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
private String url;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column excel.release_time
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
private Integer releaseTime;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column excel.search_time
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
private Integer searchTime;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column excel.created
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
private Integer created;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column excel.updated
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
private Integer updated;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column excel.deleted
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
private Integer deleted;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column excel.valid
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
private Short valid;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column excel.baidu_count
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
private Integer baiduCount;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column excel.news_count
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
private Integer newsCount;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table excel
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
private static final long serialVersionUID = 1L;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column excel.id
*
* @return the value of excel.id
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public Integer getId() {
return id;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column excel.id
*
* @param id the value for excel.id
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public void setId(Integer id) {
this.id = id;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column excel.url
*
* @return the value of excel.url
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public String getUrl() {
return url;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column excel.url
*
* @param url the value for excel.url
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public void setUrl(String url) {
this.url = url;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column excel.release_time
*
* @return the value of excel.release_time
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public Integer getReleaseTime() {
return releaseTime;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column excel.release_time
*
* @param releaseTime the value for excel.release_time
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public void setReleaseTime(Integer releaseTime) {
this.releaseTime = releaseTime;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column excel.search_time
*
* @return the value of excel.search_time
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public Integer getSearchTime() {
return searchTime;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column excel.search_time
*
* @param searchTime the value for excel.search_time
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public void setSearchTime(Integer searchTime) {
this.searchTime = searchTime;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column excel.created
*
* @return the value of excel.created
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public Integer getCreated() {
return created;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column excel.created
*
* @param created the value for excel.created
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public void setCreated(Integer created) {
this.created = created;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column excel.updated
*
* @return the value of excel.updated
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public Integer getUpdated() {
return updated;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column excel.updated
*
* @param updated the value for excel.updated
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public void setUpdated(Integer updated) {
this.updated = updated;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column excel.deleted
*
* @return the value of excel.deleted
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public Integer getDeleted() {
return deleted;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column excel.deleted
*
* @param deleted the value for excel.deleted
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public void setDeleted(Integer deleted) {
this.deleted = deleted;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column excel.valid
*
* @return the value of excel.valid
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public Short getValid() {
return valid;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column excel.valid
*
* @param valid the value for excel.valid
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public void setValid(Short valid) {
this.valid = valid;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column excel.baidu_count
*
* @return the value of excel.baidu_count
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public Integer getBaiduCount() {
return baiduCount;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column excel.baidu_count
*
* @param baiduCount the value for excel.baidu_count
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public void setBaiduCount(Integer baiduCount) {
this.baiduCount = baiduCount;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column excel.news_count
*
* @return the value of excel.news_count
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public Integer getNewsCount() {
return newsCount;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column excel.news_count
*
* @param newsCount the value for excel.news_count
*
* @mbggenerated Sun Mar 19 15:08:46 CST 2017
*/
public void setNewsCount(Integer newsCount) {
this.newsCount = newsCount;
}
}<file_sep>/src/main/resources/config/config.properties
weixin_appid=wx9439cbf94f9235f0
weixin_appsecret=<KEY>
weixin_mch_id=1254782501
weixin_key=<KEY>
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/HaiCategoryChildren.java
package com.ehais.figoarticle.model;
import java.util.List;
public class HaiCategoryChildren extends HaiCategory {
private static final long serialVersionUID = 142326890709229740L;
private List<HaiCategory> children;
public List<HaiCategory> getChildren() {
return children;
}
public void setChildren(List<HaiCategory> children) {
this.children = children;
}
}
<file_sep>/eh-common/src/main/java/org/ehais/service/verifyCodeComfirm.java
package org.ehais.service;
import javax.servlet.http.HttpServletRequest;
import org.ehais.tools.ReturnObject;
import org.springframework.ui.ModelMap;
public interface verifyCodeComfirm {
ReturnObject<?> confirmCode(HttpServletRequest request);
}<file_sep>/eh-common/src/main/java/org/ehais/util/Environments.java
package org.ehais.util;
/**
* 环境变量的配置
* @author lgj628
*
*/
public class Environments {
private Integer storeId;
public Integer getStoreId() {
return storeId;
}
public void setStoreId(Integer storeId) {
this.storeId = storeId;
}
}
<file_sep>/school-weixin/src/main/java/com/ehais/junit/HrApiJunit.java
package com.ehais.junit;
import java.util.HashMap;
import java.util.Map;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.SignUtil;
import org.ehais.util.httpPostAndGet;
import org.junit.Test;
import junit.framework.TestCase;
public class HrApiJunit{
private String web_url = "http://gzhmt.ehais.com";
private String timestamp = String.valueOf(System.currentTimeMillis());
private String appkey = "swetXsdfWEertr";
private String secret = "SDfs1224WEdsdf";
@Test
public void position(){
try{
Map<String, String> paramsMap = new HashMap<String, String>();
paramsMap.put("appkey", appkey);
paramsMap.put("version", "v1.0");
paramsMap.put("timestamp", timestamp);
paramsMap.put("page", "1");
paramsMap.put("len", "10");
paramsMap.put("store_id", "1");
String sign = SignUtil.getSignWS(paramsMap,secret);
paramsMap.put("sign", sign);
String req = EHttpClientUtil.httpPost(web_url+"/position", paramsMap);
System.out.println("请求返回:"+req);
}catch(Exception e){
e.printStackTrace();
}
}
@Test
public void verifycode(){
try{
Map<String, String> paramsMap = new HashMap<String, String>();
paramsMap.put("appkey", appkey);
paramsMap.put("version", "v1.0");
paramsMap.put("timestamp", timestamp);
paramsMap.put("page", "1");
paramsMap.put("len", "10");
paramsMap.put("store_id", "1");
String sign = SignUtil.getSignWS(paramsMap,secret);
paramsMap.put("sign", sign);
httpPostAndGet hpg=new httpPostAndGet();
System.out.println("请求返回:");
hpg.sendGet(web_url+"/getVerifyCode");
}catch(Exception e){
e.printStackTrace();
}
}
}
<file_sep>/FigoShop/src/main/resources/config/config-hkdeploy.properties
driverClassName=com.mysql.jdbc.Driver
validationQuery=SELECT 1
jdbc_url=jdbc:mysql://127.0.0.1:3306/hqh?useUnicode=true&characterEncoding=UTF-8&zeroDateTimeBehavior=convertToNull
jdbc_username=root
jdbc_password=<PASSWORD>
<file_sep>/FigoShop/src/main/webapp/js/repair/queryData.js
function main(){
getSummary();
}
document.addEventListener('DOMContentLoaded',main);
function getSummary(){
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
listSummary(this.responseText);
}
};
xhttp.open("POST", "/Repair/querySummary", true);
// xhttp.setRequestHeader("Content-type", "application/x-www-form-urlencoded");
xhttp.send();
}
function listSummary(Data){
var dataList=JSON.parse(Data);
var summaryList=document.getElementById('summaryList');
if(dataList.length!=0){
for(var i=0;i<dataList.length;i++){
var button=document.createElement("button");
button.type="button";
button.classList.add("list-group-item");
var header=document.createElement("h4");
header.classList.add("list-group-item-heading");
header.innerHTML=dataList[i].dateStr+'===>宿舍号:'+dataList[i].userAddress;
var conentP=document.createElement("p");
conentP.classList.add("list-group-item-text");
conentP.innerHTML=dataList[i].detail;
button.appendChild(header);
button.appendChild(conentP);
summaryList.appendChild(button);
}
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/IfchicController.java
package com.ehais.figoarticle.controller;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.lang3.StringEscapeUtils;
import org.ehais.controller.CommonController;
import org.ehais.util.Bean2Utils;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.FSO;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.mapper.HaiBrandMapper;
import com.ehais.figoarticle.mapper.HaiCategoryMapper;
import com.ehais.figoarticle.mapper.HaiGoodsMapper;
import com.ehais.figoarticle.mapper.HaiGoodsUrlMapper;
import com.ehais.figoarticle.model.HaiCategory;
import com.ehais.figoarticle.model.HaiCategoryExample;
import com.ehais.figoarticle.model.HaiGoodsAttr;
import com.ehais.figoarticle.model.HaiGoodsEntity;
import com.ehais.figoarticle.model.HaiGoodsGallery;
import com.ehais.figoarticle.model.HaiGoodsUrl;
import com.ehais.figoarticle.model.HaiGoodsUrlExample;
import com.ehais.figoarticle.model.HaiGoodsWithBLOBs;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
//完成
@Controller
@RequestMapping("/ifchic")
public class IfchicController extends FigoCommonController {
private int websiteId = 8;
private static String url = "https://www.ifchic.com";
@ResponseBody
@RequestMapping("/brand")
public String brand(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
return "";
}
@ResponseBody
@RequestMapping("/category")
public String category(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
this.categorys(request, url);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private void categorys(HttpServletRequest request,String websiteUrl){
String result = "";
try {
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), websiteUrl);
// result = PythonUtil.python("C:/Users/cjc/Desktop/eh-project/FigoShop/getAjaxWeb.py", websiteUrl);
// result = FSO.ReadFileName("E:/temp/IFCHIC.htm");
// Document doc = Jsoup.connect(websiteUrl).get();
Document doc = Jsoup.parse(result);
//得到菜单项
Element menu = doc.getElementById("menu");
//得到菜单的子列表
Elements menuLi = menu.select(">li");
// System.out.println(menuLi);
//删掉第一、二个元素
menuLi.remove(1);
menuLi.remove(0);
// System.out.println("===========================********");
// System.out.println("===========================********");
// System.out.println(menuLi);
List<HaiCategory> list = new ArrayList<HaiCategory>();
String parentHref = "";
for (Element element : menuLi) {
Element parentA = element.select(">a").first();
System.out.println(parentA.text());
Element adtm_sub = element.select(">div.adtm_sub").first();
if(adtm_sub == null)
continue;
Elements childrenLi = adtm_sub.getElementsByTag("li");
if(childrenLi == null)
continue;
parentHref = parentA.attr("href");
if(parentHref.indexOf("http") < 0)
parentHref = url + parentHref;
HaiCategory cat = new HaiCategory();
cat.setCatName(parentA.text());
cat.setCategoryUrl(parentHref);
cat.setIsShow(true);
cat.setWebsiteId(websiteId);
List<HaiCategory> catList = new ArrayList<HaiCategory>();
for (Element element2 : childrenLi) {
Elements childrenA = element2.getElementsByTag("a");
// System.out.println("\nchildrenA" + childrenA + "childrenA\n");
if(childrenA == null)
continue;
if(childrenA.text() == null || childrenA.text().equals(""))
continue;
for (Element element3 : childrenA) {
System.out.println("======" + element3.text());
HaiCategory cat2 = new HaiCategory();
cat2.setCatName(element3.text());
cat2.setWebsiteId(websiteId);
// System.out.println(element3.attr("href") + element3.attr("href").indexOf("http"));
if((element3.attr("href").indexOf("http")) < 0)
cat2.setCategoryUrl(url + element3.attr("href"));
else
cat2.setCategoryUrl(element3.attr("href"));
// System.out.println(cat.getCategoryUrl());
cat2.setIsShow(true);
catList.add(cat2);
}
cat.setChildren(catList);
}
list.add(cat);
}
System.out.println("==========================================");
System.out.println("==========================================");
JSONArray arr = JSONArray.fromObject(list);
System.out.println(arr.toString());
// System.out.println(element.html());
System.out.println("==========================================");
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/category";
// String api = "http://localhost:8087/api/category";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@ResponseBody
@RequestMapping("/url")
public String url(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
c.andCategoryUrlLike(url+"%");
List<HaiCategory> listCategory = haiCategoryMapper.selectByExample(example);
for (HaiCategory haiCategory : listCategory) {
System.out.println(haiCategory.getCategoryUrl());
this.goodsUrl(request, haiCategory.getCategoryUrl(), haiCategory.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsUrl(HttpServletRequest request,String goodsurl,Integer catId){
System.out.println("请求地址:"+goodsurl);
String result = "";
try{
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
// result = PythonUtil.python("D:/workspace_jee/figoarticle/src/main/webapp/getAjaxWeb.py", goodsurl);
// result = FSO.ReadFileName("E:/temp/IFCHIC.htm");
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(goodsurl).get();
Element product_list = doc.getElementById("product_list");
Elements product_li = product_list.select(">li");
List<String> list = new ArrayList<String>();
for (Element element : product_li) {
Element product_content = element.getElementsByClass("product_content").first();
Element product_a = product_content.select(">a").first();
System.out.println(product_a.attr("href"));
list.add(product_a.attr("href"));
}
JSONArray arr = JSONArray.fromObject(list);
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("catId", catId.toString());
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/url";
// String api = "http://localhost:8087/api/url";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
Element pagination_next = doc.getElementById("pagination_next");
if(pagination_next != null){
Element next_a = pagination_next.getElementsByTag("a").first();
if(next_a != null){
String href_a = next_a.attr("href");
this.goodsUrl(request, url + href_a, catId);
System.out.println(url + href_a);
}
}
}catch(Exception e){
e.printStackTrace();
}
return result;
}
@ResponseBody
@RequestMapping("/goodsAll")
public String goodsAll(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiGoodsUrlExample example = new HaiGoodsUrlExample();
HaiGoodsUrlExample.Criteria c = example.createCriteria();
c.andGoodsUrlLike(url+"%");
c.andFinishEqualTo(false);
List<HaiGoodsUrl> listGoodsUrl = haiGoodsUrlMapper.selectByExample(example);
for (HaiGoodsUrl haiGoodsUrl : listGoodsUrl) {
goodsModel(request,haiGoodsUrl.getGoodsUrl(),haiGoodsUrl.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsModel(HttpServletRequest request , String goodsurl ,Integer catId){
System.out.println(goodsurl);
String result = "";
HaiGoodsEntity entity = new HaiGoodsEntity();
HaiGoodsWithBLOBs goods = new HaiGoodsWithBLOBs();
List<HaiGoodsGallery> goodsGalleryList = new ArrayList<HaiGoodsGallery>();
//
List<HaiGoodsAttr> goodsAttrList = new ArrayList<HaiGoodsAttr>();
try{
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
// result = PythonUtil.python("D:/workspace_jee/figoarticle/src/main/webapp/getAjaxWeb.py", goodsurl);
// result = FSO.ReadFileName("E:/temp/IFCHIC.htm");
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(goodsurl).get();
Element primary_block = doc.getElementById("primary_block");
String goodsName = primary_block.select("h1.product_name").first().text();
goods.setGoodsName(goodsName);
goods.setCatId(catId);
goods.setGoodsUrl(goodsurl);
goods.setWebsiteId(websiteId);
Element price = primary_block.select("p.price").first();
Integer shopPrice = Float.valueOf(price.select(">span").select(">span").text().trim()).intValue() * 100;
String currency = price.select(">span").select(">meta").attr("content");
goods.setShopPrice(shopPrice);
goods.setCurrency(currency);
Element productRightAjax = doc.getElementById("productRightAjax");
Element color_attr = productRightAjax.select(">div").first();
HaiGoodsAttr goodsColor = new HaiGoodsAttr();
goodsColor.setAttrValue(color_attr.text());
goodsColor.setAttrType("color");
goodsColor.setAttrPrice(shopPrice.toString());
goodsAttrList.add(goodsColor);
Elements select_attr = productRightAjax.select("div.category").select("select.form-control").first().getElementsByTag("option");
for (Element element : select_attr) {
if(element.attr("value") != null && !element.attr("value").equals("")){
HaiGoodsAttr goodsAttr = new HaiGoodsAttr();
goodsAttr.setAttrValue(element.text());
goodsAttr.setAttrType("size");
goodsAttr.setAttrPrice(shopPrice.toString());
goodsAttrList.add(goodsAttr);
}
}
Element viewNotes = doc.getElementById("viewNotes");
goods.setGoodsDesc(viewNotes.html());
Element productImgBox = primary_block.select("article.productImgBox").first();
Elements section = productImgBox.select("section");
for (Element element : section) {
Element gImg = element.getElementsByTag("img").first();
String img = gImg.attr("src");
// System.out.println(img);
HaiGoodsGallery gallery = new HaiGoodsGallery();
gallery.setThumbUrl(img);
gallery.setImgUrl(img);
gallery.setImgOriginal(img);
goodsGalleryList.add(gallery);
}
if(goodsGalleryList.size() > 0){
HaiGoodsGallery gallery = goodsGalleryList.get(0);
goods.setGoodsThumb(gallery.getThumbUrl());
goods.setGoodsImg(gallery.getImgUrl());
goods.setOriginalImg(gallery.getImgOriginal());
}
// Bean2Utils.printEntity(goods);
entity.setGoods(goods);
entity.setGoodsAttrList(goodsAttrList);
entity.setGoodsGalleryList(goodsGalleryList);
JSONObject jsonObject = JSONObject.fromObject(entity);
System.out.println(jsonObject.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", jsonObject.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/goodsAttr";
// String api = "http://localhost:8087/api/goods";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
//单商品的地址请求
@ResponseBody
@RequestMapping("/getGoodsUrl")
public String getGoodsUrl(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "catId", required = true) Integer catId,
@RequestParam(value = "goodsurl", required = true) String goodsurl
){
try{
return goodsModel(request,goodsurl,catId);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
public static void main(String[] args) throws Exception {
String goodsurl = "https://www.ifchic.com/110-new-arrivals";
IfchicController ac = new IfchicController();
// ac.goodsModel(url,1);
ac.categorys(null, goodsurl);
// ac.goodsUrl(null, goodsurl,119);
// goodsurl = "https://www.ifchic.com/rachel-comey/3167-ticklers-high-rise-jeans-dirty-white.html";
// ac.goodsModel(null, goodsurl, 119);
}
}
<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/service/impl/ScrcServiceImpl.java
package com.ehais.hrlucene.service.impl;
import java.util.ArrayList;
import java.util.List;
import org.ehais.tools.ReturnObject;
import org.ehais.util.EHtmlUnit;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.nodes.Node;
import org.jsoup.select.Elements;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.ehais.hrlucene.model.HaiHrPosition;
import com.ehais.hrlucene.model.HaiHrPositionWithBLOBs;
import com.ehais.hrlucene.service.HrPositionService;
import com.ehais.hrlucene.service.ScrcService;
import net.sf.json.JSONObject;
/**
* @author stephen
*
*/
@Service("ScrcService")
public class ScrcServiceImpl implements ScrcService {
@Autowired
private HrPositionService hrPositionService;
private String scURL="http://www.scrc168.com";
private int count=1;
//private String scURL="http://www.baidu.com";
public ReturnObject rm = new ReturnObject();
public void Loadrc(String positionURL) throws Exception{
Document doc=null;
try{
System.out.println("executing Loadrc...");
List<String> hrefList=new ArrayList<String>();
System.out.println("Requesting html string....");
String htmlContent=null;
if(positionURL==null){
htmlContent=EHtmlUnit.httpUnitRequest(this.scURL+"/PersonalJobs/jobsList.aspx?SearchPost=ContetAll&Keywords=");
}else{
htmlContent=EHtmlUnit.httpUnitRequest(positionURL);
}
System.out.println("Got the HTML String,parsing....");
doc=Jsoup.parse(htmlContent);
}catch(Exception e){
System.out.println("Exception Occured");
this.rm.setCode(-1);
this.rm.setMsg("Exception Occured when getting the html content.\n"+e.getStackTrace());
return ;
}
Element JobListDiv;
try{
System.out.println("Parsing Completed.Finding the target....");
Elements JobListParentDivs=doc.getElementsByClass("job_left");
JobListDiv=(Element)JobListParentDivs.get(0).childNodes().get(3); //target reach
System.out.println("Target Reached");
}catch(Exception e){
System.out.println("Exception Occured");
this.rm.setCode(-2);
this.rm.setMsg("Exception Occured when finding the target\nExcetion: ."+e.getStackTrace());
return ;
}
int first = 1;
int childnodesnum=JobListDiv.childNodeSize();
for(Node tmp:JobListDiv.childNodes()){
Element JobDiv;
try{
JobDiv=(Element)tmp;
}catch(Exception e){
continue;
}
if (first == 1){
first = 0;
continue;
}
String DivClass=JobDiv.attr("class");
if(DivClass.indexOf("job_box3") !=-1 || DivClass.indexOf("pagination")!=-1){
break;
}
Elements t=JobDiv.getElementsByTag("li");
t=t.get(0).getElementsByTag("a");
String hrefs=t.get(0).attr("href");
hrefs=this.scURL + hrefs;
getPositionDetail(hrefs);
System.out.println("Got "+count+"!!!!!!!!!!!!!");
count++;
}
Elements buttons=doc.getElementsByClass("page-bottom");
Elements aTaginbutton=buttons.get(0).getElementsByTag("a");
for(Element aTag:aTaginbutton){
if(aTag.text().equals("下一页")){
String nextPagehref=aTag.attr("href");
Loadrc(this.scURL+nextPagehref);
}
}
}
public void getPositionDetail(String subURL) throws Exception{
//Getting the Html content
String htmlContent=null;
try{
htmlContent=EHtmlUnit.httpUnitRequest(subURL);
}catch(Exception e){
System.out.println("(positionDetail)Exception Occured");
this.rm.setCode(-1);
this.rm.setMsg("Exception Occured when getting the html content.\n"+e.getStackTrace());
return ;
}
Document doc=null;
Element JobDetailDiv;
try{
doc=Jsoup.parse(htmlContent);
Elements JobDetailDivs=doc.getElementsByClass("wrap");
JobDetailDiv=JobDetailDivs.get(2);
}catch(Exception e){
System.out.println("(positionDetail)Exception Occured");
this.rm.setCode(-2);
this.rm.setMsg("Exception Occured when finding the target\nExcetion: "+e.getStackTrace());
return ;
}
String companyName=null;
String companyIndustry=null;
String companyNature=null;
String companyScale=null;
try{
Elements allLi=JobDetailDiv.getElementsByTag("li");
companyName=allLi.get(0).text();
companyIndustry=allLi.get(5).text();
companyNature=allLi.get(4).text();
companyScale=allLi.get(3).text();
}catch(Exception e){
this.rm.setCode(-3);
this.rm.setMsg("Exception Occured when getting the infomation\nExcetion: "+e.getStackTrace());
return ;
}
String start_date = null;
String end_date = null;
String headcount = null;
String profession = null;
String degreelevel = null;
String workcity = null;
String salary = null;
String fulltime = null;
String heightbody = null;
String workyears = null;
String gender = null;
String workages = null;
String technicalpost = null;
String language = null;
String positionName = null;
String detailrequirement = null;
String issueTime = null;
try{
Elements JobDivs=doc.getElementsByClass("job_detailed");
Elements PositionNameh1s=JobDivs.get(0).getElementsByTag("h1");
positionName = PositionNameh1s.get(0).text();
detailrequirement=JobDivs.get(0).text();
int startpoint=detailrequirement.indexOf("职位描述");
detailrequirement=detailrequirement.substring(startpoint+4);
Elements PositionDetailLis=doc.getElementsByClass("infor_bg2");
int choice=1;
for(Element Li:PositionDetailLis){
switch(choice){
case 1:{
System.out.println("enter case 1");
start_date=Li.text();
choice ++;
}break;
case 2:{
System.out.println("enter case 2");
degreelevel=Li.text();
choice ++;
}break;
case 3:{
System.out.println("enter case 3");
workyears=Li.text();
choice ++;
}break;
case 4:{
System.out.println("enter case 4");
end_date=Li.text();
choice ++;
}break;
case 5:{
System.out.println("enter case 5");
workcity=Li.text();
choice ++;
}break;
case 6:{
System.out.println("enter case 6");
gender=Li.text();
choice ++;
}break;
case 7:{
System.out.println("enter case 7");
headcount=Li.text();
choice ++;
}break;
case 8:{
System.out.println("enter case 8");
salary=Li.text();
choice ++;
}break;
case 9:{
System.out.println("enter case 9");
workages=Li.text();
choice ++;
}break;
case 10:{
System.out.println("enter case 10");
profession=Li.text();
choice ++;
}break;
case 11:{
System.out.println("enter case 11");
fulltime=Li.text();
choice ++;
}break;
case 12:{
System.out.println("enter case 12");
technicalpost=Li.text();
choice ++;
}break;
case 13:{
System.out.println("enter case 13");
choice ++;
}break;
case 14:{
System.out.println("enter case 14");
heightbody=Li.text();
choice ++;
}break;
case 15:{
System.out.println("enter case 15");
language=Li.text();
choice ++;
}break;
default:{
System.out.println("enter the default case..");
}break;
}
}
}catch(Exception e){
this.rm.setCode(-4);
this.rm.setMsg("Exception Occured when getting the position detail\nExcetion: "+e.getStackTrace());
return ;
}
String CompanyDetailhref=null;
String companyDetail = null;
String companyWebsite = null;
String contactperson = null;
String tel=null;
String companyAddress = null;
String companyEmail = null;
String companyZip = null;
try{
Elements hrefUL=doc.getElementsByClass("view_box1");
Elements allATaginhrefUL=hrefUL.get(0).getElementsByTag("a");
String Companyhref=allATaginhrefUL.get(0).attr("href");
String Companyhtml=EHtmlUnit.httpUnitRequest(this.scURL+"/PersonalJobs/"+Companyhref);
Document CompanyDoc=Jsoup.parse(Companyhtml);
Elements CompanyDetailDivs=CompanyDoc.getElementsByClass("job_detailed");
companyDetail=CompanyDetailDivs.get(0).text();
Elements ComypanyContact_table=CompanyDoc.getElementsByTag("table");
Elements CompanyContact_trs=ComypanyContact_table.get(0).getElementsByTag("tr");
for(Element tr:CompanyContact_trs){
String tdtitle=tr.getElementsByTag("td").get(0).text();
if(tdtitle.indexOf("联系人")!=-1){
contactperson=tr.getElementsByTag("td").get(1).text();
}
else if(tdtitle.indexOf("联系电话")!=-1){
tel=tr.getElementsByTag("td").get(1).text();
}
else if(tdtitle.indexOf("电子邮箱")!=-1){
companyEmail=tr.getElementsByTag("td").get(1).text();
}
else if(tdtitle.indexOf("单位网址")!=-1){
companyWebsite=tr.getElementsByTag("td").get(1).text();
}
else if(tdtitle.indexOf("单位地址")!=-1){
companyAddress=tr.getElementsByTag("td").get(1).text();
}
else if(tdtitle.indexOf("邮政编码")!=-1){
companyZip=tr.getElementsByTag("td").get(1).text();
}
}
}catch(Exception e){
this.rm.setCode(-5);
this.rm.setMsg("Exception Occured when getting the company detail\nExcetion: "+e.getStackTrace());
return ;
}
HaiHrPositionWithBLOBs position = new HaiHrPositionWithBLOBs();
position.setPositionName(positionName);
position.setHeadcount(headcount); //招聘人数
position.setDegreelevel(degreelevel); //学位要求
position.setSalary(salary); //工资
position.setWorkyears(workyears); //工作经验
position.setWorkcity(workcity); //工作地点
position.setFulltime(fulltime); //全职
position.setProfession(profession); //专长
position.setHeightbody(heightbody); //身高
position.setWorkages(workages); //工作年龄
//position.setCensus(census); //户籍
position.setGender(gender); //性别
position.setTechnicalpost(technicalpost); //职称
position.setLanguage(language); //语言
position.setIssueTime(issueTime); //招聘起止时间
position.setDetailrequirement(detailrequirement); //要求详情
position.setCompanyName(companyName); //公司名称
position.setCompanyNature(companyNature); //公司性质
position.setCompanyScale(companyScale); //公司规模
position.setCompanyIndustry(companyIndustry); //公司行业
position.setCompanyDetail(companyDetail); //公司详情
position.setTel(tel); //电话
position.setContactperson(contactperson); //联系人
position.setCompanyEmail(companyEmail); //公司邮箱
position.setCompanyWebsite(companyWebsite); //公司网站
position.setCompanyAddress(companyAddress); //公司地址
position.setHrSource("四川人才网");
position.setHrPositionUrl(subURL);
// to database.
//HrPositionService hrPositionService = new ;
ReturnObject<HaiHrPosition> rm = hrPositionService.SaveHrPosition(position);
JSONObject obj = JSONObject.fromObject(rm);
System.out.println(obj.toString());
}
public static void main(String [] argv) throws Exception{
ScrcServiceImpl scrcServiceImpl=new ScrcServiceImpl();
scrcServiceImpl.Loadrc(null);
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/HaiGoodsCate.java
package com.ehais.figoarticle.model;
import java.io.Serializable;
import java.math.BigDecimal;
import java.util.Date;
public class HaiGoodsCate implements Serializable {
private Long goodsId;
private Integer catId;
private String calculate;
private Integer shopPrice;
public Long getGoodsId() {
return goodsId;
}
public void setGoodsId(Long goodsId) {
this.goodsId = goodsId;
}
public Integer getCatId() {
return catId;
}
public void setCatId(Integer catId) {
this.catId = catId;
}
public String getCalculate() {
return calculate;
}
public void setCalculate(String calculate) {
this.calculate = calculate;
}
public Integer getShopPrice() {
return shopPrice;
}
public void setShopPrice(Integer shopPrice) {
this.shopPrice = shopPrice;
}
}<file_sep>/src/main/resources/ehproject.sql
/*
Navicat MySQL Data Transfer
Source Server : 本地
Source Server Version : 50631
Source Host : 127.0.0.1:3306
Source Database : ehproject
Target Server Type : MYSQL
Target Server Version : 50631
File Encoding : 65001
Date: 2016-11-11 17:49:33
*/
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- Table structure for `hai_account_log`
-- ----------------------------
DROP TABLE IF EXISTS `hai_account_log`;
CREATE TABLE `hai_account_log` (
`log_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`user_id` mediumint(8) unsigned NOT NULL,
`user_money` decimal(10,2) NOT NULL,
`frozen_money` decimal(10,2) NOT NULL,
`rank_points` mediumint(9) NOT NULL,
`pay_points` mediumint(9) NOT NULL,
`change_time` int(10) unsigned NOT NULL,
`change_desc` varchar(255) NOT NULL,
`change_type` tinyint(3) unsigned NOT NULL,
PRIMARY KEY (`log_id`),
KEY `user_id` (`user_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_account_log
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_ad`
-- ----------------------------
DROP TABLE IF EXISTS `hai_ad`;
CREATE TABLE `hai_ad` (
`ad_id` int(5) unsigned NOT NULL AUTO_INCREMENT,
`position_id` smallint(5) unsigned NOT NULL DEFAULT '0',
`media_type` tinyint(3) unsigned NOT NULL DEFAULT '0',
`ad_name` varchar(60) NOT NULL DEFAULT '',
`ad_link` varchar(255) NOT NULL DEFAULT '',
`ad_code` text NOT NULL,
`link_man` varchar(60) NOT NULL DEFAULT '',
`link_email` varchar(60) NOT NULL DEFAULT '',
`link_phone` varchar(60) NOT NULL DEFAULT '',
`click_count` mediumint(8) unsigned NOT NULL DEFAULT '0',
`enabled` tinyint(3) unsigned NOT NULL DEFAULT '1',
`store_id` int(11) NOT NULL DEFAULT '0',
`start_time` date DEFAULT NULL,
`end_time` date DEFAULT NULL,
`nav_id` int(11) DEFAULT NULL COMMENT '用于关联到导航栏下面的广告位',
`sort` int(2) DEFAULT '0',
`is_mobile` smallint(1) DEFAULT '0' COMMENT '是否手机使用',
PRIMARY KEY (`ad_id`),
KEY `position_id` (`position_id`),
KEY `enabled` (`enabled`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_ad
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_admin_action`
-- ----------------------------
DROP TABLE IF EXISTS `hai_admin_action`;
CREATE TABLE `hai_admin_action` (
`action_id` tinyint(3) unsigned NOT NULL AUTO_INCREMENT,
`parent_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
`action_code` varchar(20) NOT NULL DEFAULT '',
`relevance` varchar(20) NOT NULL DEFAULT '',
PRIMARY KEY (`action_id`),
KEY `parent_id` (`parent_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_admin_action
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_admin_log`
-- ----------------------------
DROP TABLE IF EXISTS `hai_admin_log`;
CREATE TABLE `hai_admin_log` (
`log_id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`log_time` int(10) unsigned NOT NULL DEFAULT '0',
`user_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
`log_info` varchar(255) NOT NULL DEFAULT '',
`ip_address` varchar(15) NOT NULL DEFAULT '',
PRIMARY KEY (`log_id`),
KEY `log_time` (`log_time`),
KEY `user_id` (`user_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_admin_log
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_admin_message`
-- ----------------------------
DROP TABLE IF EXISTS `hai_admin_message`;
CREATE TABLE `hai_admin_message` (
`message_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`sender_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
`receiver_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
`sent_time` int(11) unsigned NOT NULL DEFAULT '0',
`read_time` int(11) unsigned NOT NULL DEFAULT '0',
`readed` tinyint(1) unsigned NOT NULL DEFAULT '0',
`deleted` tinyint(1) unsigned NOT NULL DEFAULT '0',
`title` varchar(150) NOT NULL DEFAULT '',
`message` text NOT NULL,
PRIMARY KEY (`message_id`),
KEY `sender_id` (`sender_id`,`receiver_id`),
KEY `receiver_id` (`receiver_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_admin_message
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_admin_user`
-- ----------------------------
DROP TABLE IF EXISTS `hai_admin_user`;
CREATE TABLE `hai_admin_user` (
`admin_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`user_name` varchar(60) NOT NULL DEFAULT '',
`email` varchar(60) NOT NULL DEFAULT '',
`password` varchar(32) NOT NULL DEFAULT '',
`ec_salt` varchar(10) DEFAULT NULL,
`add_time` int(11) DEFAULT '0',
`last_login` int(11) DEFAULT '0',
`last_ip` varchar(15) DEFAULT '',
`action_list` text,
`nav_list` text,
`lang_type` varchar(50) DEFAULT '',
`agency_id` smallint(5) unsigned DEFAULT NULL,
`business_id` smallint(5) unsigned DEFAULT '0',
`todolist` longtext,
`store_id` int(10) DEFAULT '0',
`project_folder` varchar(20) DEFAULT NULL COMMENT '使用文件夹',
PRIMARY KEY (`admin_id`),
KEY `user_name` (`user_name`),
KEY `agency_id` (`agency_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_admin_user
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_adsense`
-- ----------------------------
DROP TABLE IF EXISTS `hai_adsense`;
CREATE TABLE `hai_adsense` (
`from_ad` smallint(5) NOT NULL DEFAULT '0',
`referer` varchar(255) NOT NULL DEFAULT '',
`clicks` int(10) unsigned NOT NULL DEFAULT '0',
KEY `from_ad` (`from_ad`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_adsense
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_ad_custom`
-- ----------------------------
DROP TABLE IF EXISTS `hai_ad_custom`;
CREATE TABLE `hai_ad_custom` (
`ad_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`ad_type` tinyint(1) unsigned NOT NULL DEFAULT '1',
`ad_name` varchar(60) DEFAULT NULL,
`add_time` int(10) unsigned NOT NULL DEFAULT '0',
`content` mediumtext,
`url` varchar(255) DEFAULT NULL,
`ad_status` tinyint(3) unsigned NOT NULL DEFAULT '0',
`store_id` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`ad_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_ad_custom
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_ad_position`
-- ----------------------------
DROP TABLE IF EXISTS `hai_ad_position`;
CREATE TABLE `hai_ad_position` (
`position_id` tinyint(3) unsigned NOT NULL AUTO_INCREMENT,
`position_code` varchar(20) DEFAULT NULL COMMENT '识别码',
`position_name` varchar(60) NOT NULL DEFAULT '',
`ad_width` smallint(5) unsigned NOT NULL DEFAULT '0',
`ad_height` smallint(5) unsigned NOT NULL DEFAULT '0',
`position_desc` varchar(255) NOT NULL DEFAULT '',
`position_style` text,
`store_id` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`position_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_ad_position
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_affiliate_log`
-- ----------------------------
DROP TABLE IF EXISTS `hai_affiliate_log`;
CREATE TABLE `hai_affiliate_log` (
`log_id` mediumint(8) NOT NULL AUTO_INCREMENT,
`order_id` mediumint(8) NOT NULL,
`time` int(10) NOT NULL,
`user_id` mediumint(8) NOT NULL,
`user_name` varchar(60) DEFAULT NULL,
`money` decimal(10,2) NOT NULL DEFAULT '0.00',
`point` int(10) NOT NULL DEFAULT '0',
`separate_type` tinyint(1) NOT NULL DEFAULT '0',
PRIMARY KEY (`log_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_affiliate_log
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_agency`
-- ----------------------------
DROP TABLE IF EXISTS `hai_agency`;
CREATE TABLE `hai_agency` (
`agency_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`agency_name` varchar(255) NOT NULL,
`agency_desc` text NOT NULL,
`legal_person` varchar(20) DEFAULT NULL COMMENT '法人',
`linkman` varchar(20) DEFAULT NULL COMMENT '联系人',
`tel` varchar(15) DEFAULT NULL COMMENT '电话',
`fax` varchar(15) DEFAULT NULL COMMENT '传真',
`email` varchar(200) DEFAULT NULL COMMENT '邮箱',
`address` varchar(200) DEFAULT NULL COMMENT '联系地址',
`longitude` decimal(15,6) DEFAULT NULL COMMENT '经度',
`latitude` decimal(15,6) DEFAULT NULL COMMENT '纬度',
`void` enum('1','0') DEFAULT '1',
`logo` varchar(200) DEFAULT NULL COMMENT 'logo',
`website` varchar(100) DEFAULT NULL COMMENT '网址',
`store_id` int(11) DEFAULT NULL,
`pinyin` varchar(200) DEFAULT NULL COMMENT '拼音',
`country` smallint(2) DEFAULT NULL,
`province` smallint(2) DEFAULT NULL,
`city` smallint(2) DEFAULT NULL,
`county` smallint(2) DEFAULT NULL,
`district` smallint(2) DEFAULT NULL,
`street` smallint(2) DEFAULT NULL,
PRIMARY KEY (`agency_id`),
KEY `agency_name` (`agency_name`) USING BTREE
) ENGINE=MyISAM DEFAULT CHARSET=utf8 ROW_FORMAT=DYNAMIC;
-- ----------------------------
-- Records of hai_agency
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_alipay_result`
-- ----------------------------
DROP TABLE IF EXISTS `hai_alipay_result`;
CREATE TABLE `hai_alipay_result` (
`alipay_id` int(11) NOT NULL AUTO_INCREMENT,
`alipay_type` varchar(20) DEFAULT NULL COMMENT 'notify,return',
`out_trade_no` varchar(50) DEFAULT NULL COMMENT '商户订单号',
`trade_no` varchar(50) DEFAULT NULL COMMENT '支付宝交易号',
`trade_status` varchar(50) DEFAULT NULL COMMENT '交易状态',
`create_date` datetime DEFAULT NULL COMMENT '创建时间',
`body` text,
`buyer_email` varchar(50) DEFAULT NULL,
`buyer_id` varchar(30) DEFAULT NULL,
`discount` decimal(20,4) DEFAULT NULL,
`gmt_create` datetime DEFAULT NULL,
`gmt_logistics_modify` datetime DEFAULT NULL,
`gmt_payment` datetime DEFAULT NULL,
`is_success` varchar(10) DEFAULT NULL,
`is_total_fee_adjust` varchar(10) DEFAULT NULL,
`logistics_fee` decimal(20,4) DEFAULT NULL,
`logistics_payment` varchar(20) DEFAULT NULL,
`logistics_type` varchar(20) DEFAULT NULL,
`notify_id` varchar(150) DEFAULT NULL,
`notify_time` datetime DEFAULT NULL,
`notify_type` varchar(20) DEFAULT NULL,
`payment_type` varchar(20) DEFAULT NULL,
`price` decimal(20,4) DEFAULT NULL,
`quantity` int(10) DEFAULT NULL,
`receive_address` varchar(200) DEFAULT NULL,
`receive_mobile` varchar(20) DEFAULT NULL,
`receive_name` varchar(50) DEFAULT NULL,
`seller_actions` varchar(20) DEFAULT NULL,
`seller_email` varchar(50) DEFAULT NULL,
`seller_id` varchar(30) DEFAULT NULL,
`subject` varchar(200) DEFAULT NULL,
`total_fee` decimal(20,4) DEFAULT NULL,
`use_coupon` varchar(10) DEFAULT NULL,
`sign` varchar(50) DEFAULT NULL,
`sign_type` varchar(10) DEFAULT NULL,
`_URL_` varchar(100) DEFAULT NULL,
`remark` text,
PRIMARY KEY (`alipay_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_alipay_result
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_area_region`
-- ----------------------------
DROP TABLE IF EXISTS `hai_area_region`;
CREATE TABLE `hai_area_region` (
`shipping_area_id` smallint(5) unsigned NOT NULL DEFAULT '0',
`region_id` smallint(5) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`shipping_area_id`,`region_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_area_region
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_article`
-- ----------------------------
DROP TABLE IF EXISTS `hai_article`;
CREATE TABLE `hai_article` (
`article_id` int(8) unsigned NOT NULL AUTO_INCREMENT,
`cat_id` int(5) NOT NULL DEFAULT '0',
`code` varchar(20) NOT NULL,
`title` varchar(150) NOT NULL DEFAULT '',
`content` longtext NOT NULL,
`author` varchar(30) NOT NULL DEFAULT '',
`author_email` varchar(60) NOT NULL DEFAULT '',
`article_date` datetime DEFAULT NULL,
`keywords` varchar(255) NOT NULL DEFAULT '',
`is_open` smallint(3) unsigned NOT NULL DEFAULT '1',
`create_date` datetime NOT NULL DEFAULT '0000-00-00 00:00:00',
`file_url` varchar(255) NOT NULL DEFAULT '',
`open_type` smallint(1) unsigned NOT NULL DEFAULT '0',
`link` varchar(255) NOT NULL DEFAULT '',
`description` varchar(255) DEFAULT NULL,
`store_id` int(10) DEFAULT NULL,
`sort` smallint(5) DEFAULT NULL,
`article_images` varchar(200) DEFAULT NULL,
`video_url` varchar(200) DEFAULT NULL,
`article_type_code` varchar(20) DEFAULT NULL,
`user_id` bigint(20) DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
`article_enum` enum('company','article') DEFAULT 'article' COMMENT '列表文章还是公司资讯文章',
PRIMARY KEY (`article_id`),
KEY `cat_id` (`cat_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_article
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_article_cat`
-- ----------------------------
DROP TABLE IF EXISTS `hai_article_cat`;
CREATE TABLE `hai_article_cat` (
`cat_id` smallint(5) NOT NULL AUTO_INCREMENT,
`cat_name` varchar(255) NOT NULL DEFAULT '',
`cat_type` tinyint(1) unsigned NOT NULL DEFAULT '1',
`keywords` varchar(255) NOT NULL DEFAULT '',
`cat_desc` varchar(255) NOT NULL DEFAULT '',
`sort_order` tinyint(3) unsigned NOT NULL DEFAULT '1',
`show_in_nav` tinyint(1) unsigned NOT NULL DEFAULT '0',
`parent_id` smallint(5) unsigned NOT NULL DEFAULT '0',
`store_id` int(10) DEFAULT NULL,
`code` varchar(20) DEFAULT NULL,
`user_id` bigint(20) DEFAULT NULL,
`images` varchar(200) DEFAULT NULL,
PRIMARY KEY (`cat_id`),
KEY `cat_type` (`cat_type`),
KEY `sort_order` (`sort_order`),
KEY `parent_id` (`parent_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_article_cat
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_attribute`
-- ----------------------------
DROP TABLE IF EXISTS `hai_attribute`;
CREATE TABLE `hai_attribute` (
`attr_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`cat_id` smallint(5) unsigned NOT NULL DEFAULT '0',
`attr_code` varchar(20) DEFAULT NULL,
`attr_name` varchar(60) NOT NULL DEFAULT '',
`attr_input_type` tinyint(1) unsigned NOT NULL DEFAULT '1',
`attr_type` tinyint(1) unsigned NOT NULL DEFAULT '1',
`attr_values` text NOT NULL,
`attr_index` tinyint(1) unsigned NOT NULL DEFAULT '0',
`sort_order` tinyint(3) unsigned NOT NULL DEFAULT '0',
`is_linked` tinyint(1) unsigned NOT NULL DEFAULT '0',
`attr_group` tinyint(1) unsigned NOT NULL DEFAULT '0',
`store_id` int(11) DEFAULT '0' COMMENT '商家编号',
PRIMARY KEY (`attr_id`),
KEY `cat_id` (`cat_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_attribute
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_attribute_value`
-- ----------------------------
DROP TABLE IF EXISTS `hai_attribute_value`;
CREATE TABLE `hai_attribute_value` (
`attr_id` int(11) NOT NULL,
`attr_value` varchar(200) DEFAULT NULL,
`filter_id` int(11) DEFAULT NULL,
`store_id` int(11) DEFAULT '0'
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_attribute_value
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_auction_log`
-- ----------------------------
DROP TABLE IF EXISTS `hai_auction_log`;
CREATE TABLE `hai_auction_log` (
`log_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`act_id` mediumint(8) unsigned NOT NULL,
`bid_user` mediumint(8) unsigned NOT NULL,
`bid_price` decimal(10,2) unsigned NOT NULL,
`bid_time` int(10) unsigned NOT NULL,
PRIMARY KEY (`log_id`),
KEY `act_id` (`act_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_auction_log
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_auto_manage`
-- ----------------------------
DROP TABLE IF EXISTS `hai_auto_manage`;
CREATE TABLE `hai_auto_manage` (
`item_id` mediumint(8) NOT NULL,
`type` varchar(10) NOT NULL,
`starttime` int(10) NOT NULL,
`endtime` int(10) NOT NULL,
PRIMARY KEY (`item_id`,`type`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_auto_manage
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_back_goods`
-- ----------------------------
DROP TABLE IF EXISTS `hai_back_goods`;
CREATE TABLE `hai_back_goods` (
`rec_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`back_id` mediumint(8) unsigned DEFAULT '0',
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`product_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`product_sn` varchar(60) DEFAULT NULL,
`goods_name` varchar(120) DEFAULT NULL,
`brand_name` varchar(60) DEFAULT NULL,
`goods_sn` varchar(60) DEFAULT NULL,
`is_real` tinyint(1) unsigned DEFAULT '0',
`send_number` smallint(5) unsigned DEFAULT '0',
`goods_attr` text,
PRIMARY KEY (`rec_id`),
KEY `back_id` (`back_id`),
KEY `goods_id` (`goods_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_back_goods
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_back_order`
-- ----------------------------
DROP TABLE IF EXISTS `hai_back_order`;
CREATE TABLE `hai_back_order` (
`back_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`delivery_sn` varchar(20) NOT NULL,
`order_sn` varchar(20) NOT NULL,
`order_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`invoice_no` varchar(50) DEFAULT NULL,
`add_time` int(10) unsigned DEFAULT '0',
`shipping_id` tinyint(3) unsigned DEFAULT '0',
`shipping_name` varchar(120) DEFAULT NULL,
`user_id` mediumint(8) unsigned DEFAULT '0',
`action_user` varchar(30) DEFAULT NULL,
`consignee` varchar(60) DEFAULT NULL,
`address` varchar(250) DEFAULT NULL,
`country` smallint(5) unsigned DEFAULT '0',
`province` smallint(5) unsigned DEFAULT '0',
`city` smallint(5) unsigned DEFAULT '0',
`district` smallint(5) unsigned DEFAULT '0',
`sign_building` varchar(120) DEFAULT NULL,
`email` varchar(60) DEFAULT NULL,
`zipcode` varchar(60) DEFAULT NULL,
`tel` varchar(60) DEFAULT NULL,
`mobile` varchar(60) DEFAULT NULL,
`best_time` varchar(120) DEFAULT NULL,
`postscript` varchar(255) DEFAULT NULL,
`how_oos` varchar(120) DEFAULT NULL,
`insure_fee` decimal(10,2) unsigned DEFAULT '0.00',
`shipping_fee` decimal(10,2) unsigned DEFAULT '0.00',
`update_time` int(10) unsigned DEFAULT '0',
`suppliers_id` smallint(5) DEFAULT '0',
`status` tinyint(1) unsigned NOT NULL DEFAULT '0',
`return_time` int(10) unsigned DEFAULT '0',
`agency_id` smallint(5) unsigned DEFAULT '0',
PRIMARY KEY (`back_id`),
KEY `user_id` (`user_id`),
KEY `order_id` (`order_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_back_order
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_bonus_type`
-- ----------------------------
DROP TABLE IF EXISTS `hai_bonus_type`;
CREATE TABLE `hai_bonus_type` (
`type_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`type_name` varchar(60) NOT NULL DEFAULT '',
`type_money` decimal(10,2) NOT NULL DEFAULT '0.00',
`send_type` tinyint(3) unsigned NOT NULL DEFAULT '0',
`min_amount` decimal(10,2) unsigned NOT NULL DEFAULT '0.00',
`max_amount` decimal(10,2) unsigned NOT NULL DEFAULT '0.00',
`send_start_date` int(11) NOT NULL DEFAULT '0',
`send_end_date` int(11) NOT NULL DEFAULT '0',
`use_start_date` int(11) NOT NULL DEFAULT '0',
`use_end_date` int(11) NOT NULL DEFAULT '0',
`min_goods_amount` decimal(10,2) unsigned NOT NULL DEFAULT '0.00',
PRIMARY KEY (`type_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_bonus_type
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_booking_goods`
-- ----------------------------
DROP TABLE IF EXISTS `hai_booking_goods`;
CREATE TABLE `hai_booking_goods` (
`rec_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`user_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`email` varchar(60) NOT NULL DEFAULT '',
`link_man` varchar(60) NOT NULL DEFAULT '',
`tel` varchar(60) NOT NULL DEFAULT '',
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_desc` varchar(255) NOT NULL DEFAULT '',
`goods_number` smallint(5) unsigned NOT NULL DEFAULT '0',
`booking_time` int(10) unsigned NOT NULL DEFAULT '0',
`is_dispose` tinyint(1) unsigned NOT NULL DEFAULT '0',
`dispose_user` varchar(30) NOT NULL DEFAULT '',
`dispose_time` int(10) unsigned NOT NULL DEFAULT '0',
`dispose_note` varchar(255) NOT NULL DEFAULT '',
PRIMARY KEY (`rec_id`),
KEY `user_id` (`user_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_booking_goods
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_brand`
-- ----------------------------
DROP TABLE IF EXISTS `hai_brand`;
CREATE TABLE `hai_brand` (
`brand_id` int(11) unsigned NOT NULL AUTO_INCREMENT,
`brand_name` varchar(60) NOT NULL DEFAULT '',
`brand_logo` varchar(500) NOT NULL DEFAULT '',
`brand_desc` text NOT NULL,
`site_url` varchar(255) NOT NULL DEFAULT '',
`sort_order` smallint(3) unsigned NOT NULL DEFAULT '50',
`is_show` tinyint(1) unsigned NOT NULL DEFAULT '1',
`store_id` int(11) DEFAULT NULL,
`cat_id` int(11) DEFAULT NULL,
`recommend` varchar(200) DEFAULT NULL COMMENT '推荐类型,也作扩展信息',
PRIMARY KEY (`brand_id`),
KEY `is_show` (`is_show`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_brand
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_business`
-- ----------------------------
DROP TABLE IF EXISTS `hai_business`;
CREATE TABLE `hai_business` (
`business_id` int(11) unsigned NOT NULL AUTO_INCREMENT COMMENT '企业编号',
`user_id` int(11) DEFAULT NULL,
`business_name` varchar(200) DEFAULT NULL COMMENT '企业名称',
`sectors_id` int(11) DEFAULT NULL COMMENT '行业编号',
`legal_person` varchar(20) DEFAULT NULL COMMENT '法人',
`linkman` varchar(20) DEFAULT NULL COMMENT '联系人',
`tel` varchar(15) DEFAULT NULL COMMENT '电话',
`fax` varchar(15) DEFAULT NULL COMMENT '传真',
`email` varchar(200) DEFAULT NULL COMMENT '邮箱',
`address` varchar(200) DEFAULT NULL COMMENT '联系地址',
`longitude` decimal(8,8) DEFAULT NULL COMMENT '经度',
`latitude` decimal(8,8) DEFAULT NULL COMMENT '纬度',
`is_void` enum('1','0') DEFAULT '1',
`intro` text COMMENT '简介',
`logo` varchar(200) DEFAULT NULL COMMENT 'logo',
`website` varchar(100) DEFAULT NULL COMMENT '网址',
`store_id` int(11) DEFAULT NULL,
`pinyin` varchar(200) DEFAULT NULL COMMENT '拼音',
`country` smallint(2) DEFAULT NULL,
`province` smallint(2) DEFAULT NULL,
`city` smallint(2) DEFAULT NULL,
`county` smallint(2) DEFAULT NULL,
`district` smallint(2) DEFAULT NULL,
`street` smallint(2) DEFAULT NULL,
PRIMARY KEY (`business_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_business
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_business_data`
-- ----------------------------
DROP TABLE IF EXISTS `hai_business_data`;
CREATE TABLE `hai_business_data` (
`business_id` int(11) unsigned NOT NULL,
`click_count` int(11) DEFAULT '0' COMMENT ' 点击数',
`comment_count` int(11) DEFAULT '0' COMMENT '评论数',
`reply_count` int(11) DEFAULT '0' COMMENT '回复数',
`score_total` int(11) DEFAULT '0' COMMENT '评分总数',
`score_count` int(11) DEFAULT '0' COMMENT '评分数量',
PRIMARY KEY (`business_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_business_data
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_card`
-- ----------------------------
DROP TABLE IF EXISTS `hai_card`;
CREATE TABLE `hai_card` (
`card_id` tinyint(3) unsigned NOT NULL AUTO_INCREMENT,
`card_name` varchar(120) NOT NULL DEFAULT '',
`card_img` varchar(255) NOT NULL DEFAULT '',
`card_fee` decimal(6,2) unsigned NOT NULL DEFAULT '0.00',
`free_money` decimal(6,2) unsigned NOT NULL DEFAULT '0.00',
`card_desc` varchar(255) NOT NULL DEFAULT '',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`card_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_card
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_cart`
-- ----------------------------
DROP TABLE IF EXISTS `hai_cart`;
CREATE TABLE `hai_cart` (
`rec_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`user_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`session_id` char(32) CHARACTER SET utf8 COLLATE utf8_bin NOT NULL DEFAULT '',
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_sn` varchar(60) NOT NULL DEFAULT '',
`product_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_name` varchar(120) NOT NULL DEFAULT '',
`market_price` decimal(10,2) unsigned NOT NULL DEFAULT '0.00',
`goods_price` decimal(10,2) NOT NULL DEFAULT '0.00',
`goods_number` smallint(5) unsigned NOT NULL DEFAULT '0',
`goods_attr` text NOT NULL,
`goods_thumb` varchar(255) DEFAULT NULL,
`is_real` tinyint(1) unsigned NOT NULL DEFAULT '0',
`extension_code` varchar(30) NOT NULL DEFAULT '',
`parent_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`rec_type` tinyint(1) unsigned NOT NULL DEFAULT '0',
`is_gift` smallint(5) unsigned NOT NULL DEFAULT '0',
`is_shipping` tinyint(1) unsigned NOT NULL DEFAULT '0',
`can_handsel` tinyint(3) unsigned NOT NULL DEFAULT '0',
`goods_attr_id` varchar(255) DEFAULT '',
`goods_attr_json` text,
`goods_store_id` int(11) DEFAULT NULL COMMENT '商品所属商家编号',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`rec_id`),
KEY `session_id` (`session_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_cart
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_category`
-- ----------------------------
DROP TABLE IF EXISTS `hai_category`;
CREATE TABLE `hai_category` (
`cat_id` int(11) unsigned NOT NULL AUTO_INCREMENT,
`cat_name` varchar(90) NOT NULL DEFAULT '',
`keywords` varchar(255) NOT NULL DEFAULT '',
`sub_cat_name` varchar(50) DEFAULT NULL,
`cat_desc` varchar(255) NOT NULL DEFAULT '',
`parent_id` int(11) unsigned NOT NULL DEFAULT '0',
`sort_order` smallint(1) unsigned NOT NULL DEFAULT '0',
`template_file` varchar(50) NOT NULL DEFAULT '',
`measure_unit` varchar(15) NOT NULL DEFAULT '',
`show_in_nav` tinyint(1) NOT NULL DEFAULT '0',
`style` varchar(150) NOT NULL,
`is_show` tinyint(1) unsigned NOT NULL DEFAULT '1',
`grade` smallint(4) NOT NULL DEFAULT '0',
`filter_attr` text NOT NULL COMMENT '筛选属性编号',
`sub_parent_id` text COMMENT '子分类的编号',
`brand_id` text COMMENT '品牌编号',
`store_id` int(10) DEFAULT NULL COMMENT '商家编号',
`thumb` varchar(200) DEFAULT NULL COMMENT '缩略图',
`image` varchar(200) DEFAULT NULL COMMENT '图片',
`cat_code` varchar(20) DEFAULT NULL,
`user_id` bigint(20) DEFAULT NULL,
`recommend` varchar(20) DEFAULT NULL COMMENT '推荐类型',
PRIMARY KEY (`cat_id`),
KEY `parent_id` (`parent_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_category
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_cat_recommend`
-- ----------------------------
DROP TABLE IF EXISTS `hai_cat_recommend`;
CREATE TABLE `hai_cat_recommend` (
`cat_id` smallint(5) NOT NULL,
`recommend_type` tinyint(1) NOT NULL,
PRIMARY KEY (`cat_id`,`recommend_type`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_cat_recommend
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_chapter`
-- ----------------------------
DROP TABLE IF EXISTS `hai_chapter`;
CREATE TABLE `hai_chapter` (
`cha_id` int(11) NOT NULL AUTO_INCREMENT,
`cha_name` varchar(100) DEFAULT NULL,
`goods_id` int(11) DEFAULT NULL COMMENT '所属商品编号',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`cha_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_chapter
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_collect_goods`
-- ----------------------------
DROP TABLE IF EXISTS `hai_collect_goods`;
CREATE TABLE `hai_collect_goods` (
`rec_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`user_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`add_time` int(11) unsigned NOT NULL DEFAULT '0',
`is_attention` tinyint(1) NOT NULL DEFAULT '0',
PRIMARY KEY (`rec_id`),
KEY `user_id` (`user_id`),
KEY `goods_id` (`goods_id`),
KEY `is_attention` (`is_attention`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_collect_goods
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_comment`
-- ----------------------------
DROP TABLE IF EXISTS `hai_comment`;
CREATE TABLE `hai_comment` (
`comment_id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`comment_type` tinyint(3) unsigned NOT NULL DEFAULT '0',
`id_value` mediumint(8) unsigned NOT NULL DEFAULT '0',
`email` varchar(60) NOT NULL DEFAULT '',
`user_name` varchar(60) NOT NULL DEFAULT '',
`content` text NOT NULL,
`comment_rank` tinyint(1) unsigned NOT NULL DEFAULT '0',
`add_time` int(10) unsigned NOT NULL DEFAULT '0',
`ip_address` varchar(15) NOT NULL DEFAULT '',
`status` tinyint(3) unsigned NOT NULL DEFAULT '0',
`parent_id` int(10) unsigned NOT NULL DEFAULT '0',
`user_id` int(10) unsigned NOT NULL DEFAULT '0',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`comment_id`),
KEY `parent_id` (`parent_id`),
KEY `id_value` (`id_value`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_comment
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_company`
-- ----------------------------
DROP TABLE IF EXISTS `hai_company`;
CREATE TABLE `hai_company` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`code` varchar(20) DEFAULT NULL,
`title` varchar(20) DEFAULT NULL,
`content` text,
`store_id` int(11) DEFAULT NULL,
`parent_id` int(11) DEFAULT '0',
`summary` text COMMENT '摘要',
`keywords` varchar(200) DEFAULT NULL,
`sort` int(5) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_company
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_crons`
-- ----------------------------
DROP TABLE IF EXISTS `hai_crons`;
CREATE TABLE `hai_crons` (
`cron_id` tinyint(3) unsigned NOT NULL AUTO_INCREMENT,
`cron_code` varchar(20) NOT NULL,
`cron_name` varchar(120) NOT NULL,
`cron_desc` text,
`cron_order` tinyint(3) unsigned NOT NULL DEFAULT '0',
`cron_config` text NOT NULL,
`thistime` int(10) NOT NULL DEFAULT '0',
`nextime` int(10) NOT NULL,
`day` tinyint(2) NOT NULL,
`week` varchar(1) NOT NULL,
`hour` varchar(2) NOT NULL,
`minute` varchar(255) NOT NULL,
`enable` tinyint(1) NOT NULL DEFAULT '1',
`run_once` tinyint(1) NOT NULL DEFAULT '0',
`allow_ip` varchar(100) NOT NULL DEFAULT '',
`alow_files` varchar(255) NOT NULL,
PRIMARY KEY (`cron_id`),
KEY `nextime` (`nextime`),
KEY `enable` (`enable`),
KEY `cron_code` (`cron_code`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_crons
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_delivery_goods`
-- ----------------------------
DROP TABLE IF EXISTS `hai_delivery_goods`;
CREATE TABLE `hai_delivery_goods` (
`rec_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`delivery_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`product_id` mediumint(8) unsigned DEFAULT '0',
`product_sn` varchar(60) DEFAULT NULL,
`goods_name` varchar(120) DEFAULT NULL,
`brand_name` varchar(60) DEFAULT NULL,
`goods_sn` varchar(60) DEFAULT NULL,
`is_real` tinyint(1) unsigned DEFAULT '0',
`extension_code` varchar(30) DEFAULT NULL,
`parent_id` mediumint(8) unsigned DEFAULT '0',
`send_number` smallint(5) unsigned DEFAULT '0',
`goods_attr` text,
PRIMARY KEY (`rec_id`),
KEY `delivery_id` (`delivery_id`,`goods_id`),
KEY `goods_id` (`goods_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_delivery_goods
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_delivery_order`
-- ----------------------------
DROP TABLE IF EXISTS `hai_delivery_order`;
CREATE TABLE `hai_delivery_order` (
`delivery_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`delivery_sn` varchar(20) NOT NULL,
`order_sn` varchar(20) NOT NULL,
`order_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`invoice_no` varchar(50) DEFAULT NULL,
`add_time` int(10) unsigned DEFAULT '0',
`shipping_id` tinyint(3) unsigned DEFAULT '0',
`shipping_name` varchar(120) DEFAULT NULL,
`user_id` mediumint(8) unsigned DEFAULT '0',
`action_user` varchar(30) DEFAULT NULL,
`consignee` varchar(60) DEFAULT NULL,
`address` varchar(250) DEFAULT NULL,
`country` smallint(5) unsigned DEFAULT '0',
`province` smallint(5) unsigned DEFAULT '0',
`city` smallint(5) unsigned DEFAULT '0',
`district` smallint(5) unsigned DEFAULT '0',
`sign_building` varchar(120) DEFAULT NULL,
`email` varchar(60) DEFAULT NULL,
`zipcode` varchar(60) DEFAULT NULL,
`tel` varchar(60) DEFAULT NULL,
`mobile` varchar(60) DEFAULT NULL,
`best_time` varchar(120) DEFAULT NULL,
`postscript` varchar(255) DEFAULT NULL,
`how_oos` varchar(120) DEFAULT NULL,
`insure_fee` decimal(10,2) unsigned DEFAULT '0.00',
`shipping_fee` decimal(10,2) unsigned DEFAULT '0.00',
`update_time` int(10) unsigned DEFAULT '0',
`suppliers_id` smallint(5) DEFAULT '0',
`status` tinyint(1) unsigned NOT NULL DEFAULT '0',
`agency_id` smallint(5) unsigned DEFAULT '0',
PRIMARY KEY (`delivery_id`),
KEY `user_id` (`user_id`),
KEY `order_id` (`order_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_delivery_order
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_donation`
-- ----------------------------
DROP TABLE IF EXISTS `hai_donation`;
CREATE TABLE `hai_donation` (
`donation_id` int(11) NOT NULL AUTO_INCREMENT COMMENT '自动编辑',
`donation_name` varchar(50) DEFAULT NULL COMMENT '捐款项目名称',
`donation_type_id` int(11) DEFAULT NULL COMMENT '捐款类型编号',
`picture` varchar(200) DEFAULT NULL COMMENT '图片',
`intro` varchar(100) DEFAULT NULL COMMENT '摘要',
`content` text COMMENT '内容',
`sort` int(5) DEFAULT NULL COMMENT '排序',
`store_id` int(11) DEFAULT NULL,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
`total_amount` int(11) DEFAULT NULL COMMENT '捐款总金额',
`total_quantity` int(11) DEFAULT NULL COMMENT '捐款总人数',
PRIMARY KEY (`donation_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_donation
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_email_list`
-- ----------------------------
DROP TABLE IF EXISTS `hai_email_list`;
CREATE TABLE `hai_email_list` (
`id` mediumint(8) NOT NULL AUTO_INCREMENT,
`email` varchar(60) NOT NULL,
`stat` tinyint(1) NOT NULL DEFAULT '0',
`hash` varchar(10) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_email_list
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_email_sendlist`
-- ----------------------------
DROP TABLE IF EXISTS `hai_email_sendlist`;
CREATE TABLE `hai_email_sendlist` (
`id` mediumint(8) NOT NULL AUTO_INCREMENT,
`email` varchar(100) NOT NULL,
`template_id` mediumint(8) NOT NULL,
`email_content` text NOT NULL,
`error` tinyint(1) NOT NULL DEFAULT '0',
`pri` tinyint(10) NOT NULL,
`last_send` int(10) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_email_sendlist
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_error_log`
-- ----------------------------
DROP TABLE IF EXISTS `hai_error_log`;
CREATE TABLE `hai_error_log` (
`id` int(10) NOT NULL AUTO_INCREMENT,
`info` varchar(255) NOT NULL,
`file` varchar(100) NOT NULL,
`time` int(10) NOT NULL,
PRIMARY KEY (`id`),
KEY `time` (`time`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_error_log
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_exchange_goods`
-- ----------------------------
DROP TABLE IF EXISTS `hai_exchange_goods`;
CREATE TABLE `hai_exchange_goods` (
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`exchange_integral` int(10) unsigned NOT NULL DEFAULT '0',
`is_exchange` tinyint(1) unsigned NOT NULL DEFAULT '0',
`is_hot` tinyint(1) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`goods_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_exchange_goods
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_extend_fields`
-- ----------------------------
DROP TABLE IF EXISTS `hai_extend_fields`;
CREATE TABLE `hai_extend_fields` (
`field_id` tinyint(3) unsigned NOT NULL AUTO_INCREMENT,
`field_name` varchar(60) NOT NULL DEFAULT '',
`field_label` varchar(60) DEFAULT NULL,
`extend_table` varchar(60) NOT NULL,
`dis_order` tinyint(3) unsigned NOT NULL DEFAULT '2',
`display` tinyint(1) unsigned NOT NULL DEFAULT '1',
`type` varchar(20) NOT NULL DEFAULT '0',
`is_need` tinyint(1) unsigned NOT NULL DEFAULT '1',
`control` varchar(20) DEFAULT '0',
`prompt` varchar(200) DEFAULT NULL COMMENT '提示信息',
`length` int(11) DEFAULT '20',
`extend_value_list` text COMMENT '扩展字段列表,以逗号分开',
`store_id` int(11) DEFAULT '0',
PRIMARY KEY (`field_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_extend_fields
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_extend_value`
-- ----------------------------
DROP TABLE IF EXISTS `hai_extend_value`;
CREATE TABLE `hai_extend_value` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`field_id` int(10) unsigned NOT NULL,
`content` text NOT NULL,
`table_id` int(11) DEFAULT NULL COMMENT '对应表的编号',
`store_id` int(11) DEFAULT '0',
`field_name` varchar(50) DEFAULT NULL,
`table_name` varchar(50) DEFAULT NULL,
`user_id` int(11) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_extend_value
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_favorites`
-- ----------------------------
DROP TABLE IF EXISTS `hai_favorites`;
CREATE TABLE `hai_favorites` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) DEFAULT NULL,
`goods_id` int(11) DEFAULT NULL,
`add_time` datetime DEFAULT NULL,
`sesskey` varchar(64) DEFAULT NULL COMMENT '未登陆时的sesskey',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `goods_id` (`goods_id`),
KEY `user_id` (`user_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_favorites
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_favourable_activity`
-- ----------------------------
DROP TABLE IF EXISTS `hai_favourable_activity`;
CREATE TABLE `hai_favourable_activity` (
`act_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`act_name` varchar(255) NOT NULL,
`start_time` int(10) unsigned NOT NULL,
`end_time` int(10) unsigned NOT NULL,
`user_rank` varchar(255) NOT NULL,
`act_range` tinyint(3) unsigned NOT NULL,
`act_range_ext` varchar(255) NOT NULL,
`min_amount` decimal(10,2) unsigned NOT NULL,
`max_amount` decimal(10,2) unsigned NOT NULL,
`act_type` tinyint(3) unsigned NOT NULL,
`act_type_ext` decimal(10,2) unsigned NOT NULL,
`gift` text NOT NULL,
`sort_order` tinyint(3) unsigned NOT NULL DEFAULT '50',
PRIMARY KEY (`act_id`),
KEY `act_name` (`act_name`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_favourable_activity
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_feedback`
-- ----------------------------
DROP TABLE IF EXISTS `hai_feedback`;
CREATE TABLE `hai_feedback` (
`msg_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`parent_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`user_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`user_name` varchar(60) NOT NULL DEFAULT '',
`user_email` varchar(60) NOT NULL DEFAULT '',
`msg_title` varchar(200) NOT NULL DEFAULT '',
`msg_type` tinyint(1) unsigned NOT NULL DEFAULT '0',
`msg_status` tinyint(1) unsigned NOT NULL DEFAULT '0',
`msg_content` text NOT NULL,
`msg_time` int(10) unsigned NOT NULL DEFAULT '0',
`message_img` varchar(255) NOT NULL DEFAULT '0',
`order_id` int(11) unsigned NOT NULL DEFAULT '0',
`msg_area` tinyint(1) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`msg_id`),
KEY `user_id` (`user_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_feedback
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_forum`
-- ----------------------------
DROP TABLE IF EXISTS `hai_forum`;
CREATE TABLE `hai_forum` (
`forum_id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) DEFAULT NULL,
`goods_id` int(11) DEFAULT NULL,
`content` text,
`create_date` datetime DEFAULT NULL,
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`forum_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_forum
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_friend_link`
-- ----------------------------
DROP TABLE IF EXISTS `hai_friend_link`;
CREATE TABLE `hai_friend_link` (
`link_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`link_name` varchar(255) NOT NULL DEFAULT '',
`link_url` varchar(255) NOT NULL DEFAULT '',
`link_logo` varchar(255) NOT NULL DEFAULT '',
`show_order` tinyint(3) unsigned NOT NULL DEFAULT '50',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`link_id`),
KEY `show_order` (`show_order`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_friend_link
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods`;
CREATE TABLE `hai_goods` (
`goods_id` bigint(8) unsigned NOT NULL AUTO_INCREMENT,
`cat_id` int(5) unsigned NOT NULL DEFAULT '0',
`goods_sn` varchar(60) NOT NULL DEFAULT '',
`goods_name` varchar(120) NOT NULL DEFAULT '',
`goods_name_style` varchar(60) NOT NULL DEFAULT '+',
`click_count` int(10) unsigned NOT NULL DEFAULT '0',
`brand_id` int(5) unsigned NOT NULL DEFAULT '0',
`provider_name` varchar(100) NOT NULL DEFAULT '',
`goods_number` smallint(5) unsigned NOT NULL DEFAULT '0',
`goods_weight` int(10) unsigned NOT NULL DEFAULT '0',
`market_price` int(10) unsigned NOT NULL DEFAULT '0',
`shop_price` int(10) unsigned NOT NULL DEFAULT '0',
`cost_price` int(10) DEFAULT '0',
`promote_price` int(10) unsigned NOT NULL DEFAULT '0',
`promote_start_date` date NOT NULL DEFAULT '0000-00-00',
`promote_end_date` date NOT NULL DEFAULT '0000-00-00',
`warn_number` tinyint(3) unsigned NOT NULL DEFAULT '1',
`keywords` varchar(255) NOT NULL DEFAULT '',
`goods_brief` text NOT NULL,
`goods_desc` text NOT NULL,
`act_desc` varchar(100) DEFAULT NULL COMMENT '标题旁边的标注',
`app_desc` text COMMENT '手机端描述',
`goods_thumb` varchar(255) NOT NULL DEFAULT '',
`goods_img` varchar(255) NOT NULL DEFAULT '',
`original_img` varchar(255) NOT NULL DEFAULT '',
`is_real` tinyint(3) unsigned NOT NULL DEFAULT '1',
`extension_code` varchar(30) NOT NULL DEFAULT '',
`is_on_sale` tinyint(1) unsigned NOT NULL DEFAULT '1',
`is_alone_sale` tinyint(1) unsigned NOT NULL DEFAULT '1',
`is_shipping` tinyint(1) unsigned NOT NULL DEFAULT '0',
`integral` int(10) unsigned NOT NULL DEFAULT '0',
`add_time` int(10) unsigned NOT NULL DEFAULT '0',
`sort_order` smallint(4) unsigned NOT NULL DEFAULT '100' COMMENT '排序',
`is_delete` tinyint(1) unsigned NOT NULL DEFAULT '0',
`is_best` tinyint(1) unsigned NOT NULL DEFAULT '0',
`is_new` tinyint(1) unsigned NOT NULL DEFAULT '0',
`is_hot` tinyint(1) unsigned NOT NULL DEFAULT '0',
`is_promote` tinyint(1) unsigned NOT NULL DEFAULT '0',
`is_special` tinyint(1) NOT NULL DEFAULT '0',
`bonus_type_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
`last_update` int(10) unsigned NOT NULL DEFAULT '0',
`goods_type` smallint(5) unsigned NOT NULL DEFAULT '0',
`seller_note` varchar(255) NOT NULL DEFAULT '',
`give_integral` int(11) NOT NULL DEFAULT '-1',
`rank_integral` int(11) NOT NULL DEFAULT '-1',
`business_id` smallint(5) unsigned DEFAULT NULL,
`is_check` tinyint(1) unsigned DEFAULT NULL,
`tb_iid` varchar(40) DEFAULT NULL,
`tb_data` text,
`is_export` smallint(6) DEFAULT '0' COMMENT '0不导出,1导出',
`user_id` int(11) DEFAULT '0' COMMENT '用户编号,store_id必须为0,针对用户自己的商品',
`store_id` int(10) DEFAULT '0' COMMENT '商家的编号',
`exchangeRate` decimal(10,4) DEFAULT '0.0000' COMMENT '兑换率',
`profit` decimal(10,4) DEFAULT '0.0000' COMMENT '利益',
`shipping` decimal(10,4) DEFAULT '0.0000' COMMENT '运费',
`profitType` int(2) DEFAULT '0' COMMENT '0:乘以折扣,1:加上费用',
`currency` varchar(20) DEFAULT NULL COMMENT '货币类型',
`goods_url` varchar(500) DEFAULT NULL COMMENT '网上抓取的网址',
`tabbox` int(11) DEFAULT NULL COMMENT 'tabbox推荐商品类别',
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
PRIMARY KEY (`goods_id`),
KEY `goods_sn` (`goods_sn`),
KEY `cat_id` (`cat_id`),
KEY `last_update` (`last_update`),
KEY `brand_id` (`brand_id`),
KEY `goods_weight` (`goods_weight`),
KEY `promote_end_date` (`promote_end_date`),
KEY `promote_start_date` (`promote_start_date`),
KEY `goods_number` (`goods_number`),
KEY `sort_order` (`sort_order`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods_activity`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods_activity`;
CREATE TABLE `hai_goods_activity` (
`act_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`act_name` varchar(255) NOT NULL,
`act_desc` text NOT NULL,
`act_type` tinyint(3) unsigned NOT NULL,
`goods_id` mediumint(8) unsigned NOT NULL,
`product_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_name` varchar(255) NOT NULL,
`start_time` int(10) unsigned NOT NULL,
`end_time` int(10) unsigned NOT NULL,
`is_finished` tinyint(3) unsigned NOT NULL,
`ext_info` text NOT NULL,
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`act_id`),
KEY `act_name` (`act_name`,`act_type`,`goods_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods_activity
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods_article`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods_article`;
CREATE TABLE `hai_goods_article` (
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`article_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`admin_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`goods_id`,`article_id`,`admin_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods_article
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods_attr`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods_attr`;
CREATE TABLE `hai_goods_attr` (
`goods_attr_id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`attr_id` smallint(5) unsigned NOT NULL DEFAULT '0',
`attr_value` text NOT NULL,
`attr_price` varchar(255) NOT NULL DEFAULT '',
`taobao_vid` bigint(20) DEFAULT '0',
PRIMARY KEY (`goods_attr_id`),
KEY `goods_id` (`goods_id`),
KEY `attr_id` (`attr_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods_attr
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods_cat`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods_cat`;
CREATE TABLE `hai_goods_cat` (
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`cat_id` smallint(5) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`goods_id`,`cat_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods_cat
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods_gallery`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods_gallery`;
CREATE TABLE `hai_goods_gallery` (
`img_id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`user_id` bigint(20) DEFAULT '0',
`goods_id` bigint(20) unsigned NOT NULL DEFAULT '0',
`table_name` varchar(50) DEFAULT NULL,
`img_url` varchar(255) NOT NULL DEFAULT '',
`img_desc` varchar(255) NOT NULL DEFAULT '',
`thumb_url` varchar(255) NOT NULL DEFAULT '',
`img_original` varchar(255) NOT NULL DEFAULT '',
`gallery_type` smallint(1) DEFAULT '0' COMMENT '相册类型',
`store_id` int(11) DEFAULT '0' COMMENT '商家编号',
PRIMARY KEY (`img_id`),
KEY `goods_id` (`goods_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods_gallery
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods_type`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods_type`;
CREATE TABLE `hai_goods_type` (
`cat_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`cat_name` varchar(60) NOT NULL DEFAULT '',
`enabled` tinyint(1) unsigned NOT NULL DEFAULT '1',
`attr_group` varchar(255) NOT NULL,
`store_id` int(11) DEFAULT '0' COMMENT '商家编号',
PRIMARY KEY (`cat_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods_type
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_group_goods`
-- ----------------------------
DROP TABLE IF EXISTS `hai_group_goods`;
CREATE TABLE `hai_group_goods` (
`parent_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_price` decimal(10,2) unsigned NOT NULL DEFAULT '0.00',
`admin_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`parent_id`,`goods_id`,`admin_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_group_goods
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_guestbook`
-- ----------------------------
DROP TABLE IF EXISTS `hai_guestbook`;
CREATE TABLE `hai_guestbook` (
`guestbook_id` int(11) NOT NULL AUTO_INCREMENT,
`title` varchar(100) DEFAULT NULL,
`content` text,
`guest` varchar(50) DEFAULT NULL,
`sex` smallint(1) DEFAULT NULL,
`company` varchar(200) DEFAULT NULL,
`address` varchar(200) DEFAULT NULL,
`postcode` varchar(50) DEFAULT NULL,
`telphone` varchar(50) DEFAULT NULL,
`fax` varchar(50) DEFAULT NULL,
`mobile` varchar(50) DEFAULT NULL,
`email` varchar(50) DEFAULT NULL,
`store_id` int(11) DEFAULT NULL,
`status` smallint(1) DEFAULT NULL,
`result_message` text COMMENT '处理结果信息',
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
PRIMARY KEY (`guestbook_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_guestbook
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_job_apply`
-- ----------------------------
DROP TABLE IF EXISTS `hai_job_apply`;
CREATE TABLE `hai_job_apply` (
`mja_id` int(11) NOT NULL AUTO_INCREMENT,
`job_id` int(11) DEFAULT NULL,
`user_id` int(11) DEFAULT NULL,
`job_type` smallint(2) DEFAULT '0' COMMENT '1:模特,2:翻译',
`pass` smallint(2) DEFAULT NULL,
`remark` text COMMENT '备注信息',
`store_id` int(11) DEFAULT NULL,
`create_date` datetime DEFAULT NULL,
PRIMARY KEY (`mja_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_job_apply
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_keywords`
-- ----------------------------
DROP TABLE IF EXISTS `hai_keywords`;
CREATE TABLE `hai_keywords` (
`date` date NOT NULL DEFAULT '0000-00-00',
`searchengine` varchar(20) NOT NULL DEFAULT '',
`keyword` varchar(90) NOT NULL DEFAULT '',
`count` mediumint(8) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`date`,`searchengine`,`keyword`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_keywords
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_link_goods`
-- ----------------------------
DROP TABLE IF EXISTS `hai_link_goods`;
CREATE TABLE `hai_link_goods` (
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`link_goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`is_double` tinyint(1) unsigned NOT NULL DEFAULT '0',
`admin_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`goods_id`,`link_goods_id`,`admin_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_link_goods
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_mail_templates`
-- ----------------------------
DROP TABLE IF EXISTS `hai_mail_templates`;
CREATE TABLE `hai_mail_templates` (
`template_id` tinyint(1) unsigned NOT NULL AUTO_INCREMENT,
`template_code` varchar(30) NOT NULL DEFAULT '',
`is_html` tinyint(1) unsigned NOT NULL DEFAULT '0',
`template_subject` varchar(200) NOT NULL DEFAULT '',
`template_content` text NOT NULL,
`last_modify` int(10) unsigned NOT NULL DEFAULT '0',
`last_send` int(10) unsigned NOT NULL DEFAULT '0',
`type` varchar(10) NOT NULL,
PRIMARY KEY (`template_id`),
UNIQUE KEY `template_code` (`template_code`),
KEY `type` (`type`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_mail_templates
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_member_price`
-- ----------------------------
DROP TABLE IF EXISTS `hai_member_price`;
CREATE TABLE `hai_member_price` (
`price_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`user_rank` tinyint(3) NOT NULL DEFAULT '0',
`user_price` decimal(10,2) NOT NULL DEFAULT '0.00',
PRIMARY KEY (`price_id`),
KEY `goods_id` (`goods_id`,`user_rank`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_member_price
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_model_info`
-- ----------------------------
DROP TABLE IF EXISTS `hai_model_info`;
CREATE TABLE `hai_model_info` (
`model_id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) DEFAULT NULL,
`model_name` varchar(50) DEFAULT NULL COMMENT '模特名称',
`sex` smallint(1) DEFAULT NULL,
`age` int(4) DEFAULT NULL,
`face_path` varchar(200) DEFAULT NULL COMMENT '头像图片',
`xingzuo` varchar(100) DEFAULT NULL COMMENT '星座',
`xuexing` varchar(100) DEFAULT NULL COMMENT '血型',
`faxing` varchar(100) DEFAULT NULL COMMENT '发型',
`xueli` varchar(100) DEFAULT NULL COMMENT '学历',
`school` varchar(200) DEFAULT NULL COMMENT '毕业学校',
`model_type` varchar(100) DEFAULT NULL COMMENT '模特类型',
`city` varchar(100) DEFAULT NULL,
`xinshui` varchar(100) DEFAULT NULL COMMENT '薪水',
`self_introduction` text,
`experience` text COMMENT '经验',
`awards` text COMMENT '所获奖项',
`telephone` varchar(100) DEFAULT NULL,
`mobilephone` varchar(100) DEFAULT NULL,
`qq` varchar(100) DEFAULT NULL,
`weixin` varchar(100) DEFAULT NULL,
`email` varchar(100) DEFAULT NULL,
`company` varchar(200) DEFAULT NULL,
`company_phone` varchar(100) DEFAULT NULL,
`address` varchar(500) DEFAULT NULL,
`pass` smallint(1) DEFAULT '0' COMMENT '是否通过审核',
`store_id` int(11) DEFAULT NULL,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
PRIMARY KEY (`model_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_model_info
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_model_job`
-- ----------------------------
DROP TABLE IF EXISTS `hai_model_job`;
CREATE TABLE `hai_model_job` (
`model_job_id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) DEFAULT NULL,
`title` varchar(200) DEFAULT NULL COMMENT '标题',
`issue_date` date DEFAULT NULL COMMENT '发布日期',
`end_date` date DEFAULT NULL COMMENT '截止日期',
`city` varchar(50) DEFAULT NULL COMMENT '所在城市',
`job_type` varchar(100) DEFAULT NULL COMMENT '工作类型',
`xinshui` varchar(50) DEFAULT NULL COMMENT '薪水',
`quantity` varchar(50) DEFAULT NULL COMMENT '数量',
`sex` int(1) DEFAULT NULL COMMENT '性别',
`height` varchar(50) DEFAULT NULL COMMENT '身高',
`linkman` varchar(50) DEFAULT NULL COMMENT '联系人',
`mobilephone` varchar(50) DEFAULT NULL COMMENT '手机',
`QQ` varchar(50) DEFAULT NULL COMMENT 'QQ',
`email` varchar(50) DEFAULT NULL COMMENT '邮箱',
`job_yao_qiu` text COMMENT '招聘要求',
`pass` smallint(1) DEFAULT '0' COMMENT '是否通过审核',
`store_id` int(10) DEFAULT NULL,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
PRIMARY KEY (`model_job_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 CHECKSUM=1 DELAY_KEY_WRITE=1 ROW_FORMAT=DYNAMIC;
-- ----------------------------
-- Records of hai_model_job
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_nav`
-- ----------------------------
DROP TABLE IF EXISTS `hai_nav`;
CREATE TABLE `hai_nav` (
`id` mediumint(8) NOT NULL AUTO_INCREMENT,
`ctype` varchar(10) DEFAULT NULL,
`cid` smallint(5) unsigned DEFAULT NULL,
`name` varchar(255) NOT NULL,
`ifshow` smallint(5) DEFAULT NULL,
`vieworder` smallint(5) DEFAULT NULL,
`opennew` smallint(5) DEFAULT NULL,
`url` varchar(255) DEFAULT NULL,
`type` varchar(10) DEFAULT NULL,
`sub_nav` text COMMENT '子栏',
`store_id` int(11) DEFAULT NULL,
`className` varchar(20) DEFAULT NULL COMMENT '样式名称',
`parent_nav_id` int(10) DEFAULT '0' COMMENT '父栏目',
`thumb` varchar(200) DEFAULT NULL,
`image` varchar(200) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `type` (`type`),
KEY `ifshow` (`ifshow`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_nav
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_nav_promotion`
-- ----------------------------
DROP TABLE IF EXISTS `hai_nav_promotion`;
CREATE TABLE `hai_nav_promotion` (
`id` mediumint(8) NOT NULL AUTO_INCREMENT,
`nav_id` int(10) DEFAULT NULL COMMENT 'hai_nav的外键联接字段',
`name` varchar(255) NOT NULL,
`ifshow` tinyint(1) NOT NULL,
`vieworder` tinyint(1) NOT NULL,
`opennew` tinyint(1) NOT NULL,
`url` varchar(255) NOT NULL,
`img_url` varchar(200) DEFAULT NULL COMMENT '图片地址',
`nav_left_type` int(11) DEFAULT NULL COMMENT '图片类型',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `ifshow` (`ifshow`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_nav_promotion
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_note`
-- ----------------------------
DROP TABLE IF EXISTS `hai_note`;
CREATE TABLE `hai_note` (
`note_id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) DEFAULT NULL,
`goods_id` int(11) DEFAULT NULL,
`note` text,
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`note_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_note
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_notice`
-- ----------------------------
DROP TABLE IF EXISTS `hai_notice`;
CREATE TABLE `hai_notice` (
`id` int(11) unsigned NOT NULL AUTO_INCREMENT,
`title` varchar(50) DEFAULT NULL COMMENT '标题',
`notice` text,
`is_show` int(11) DEFAULT '1',
`start_date` date DEFAULT NULL COMMENT '生效日期',
`end_date` date DEFAULT NULL COMMENT '截止日期',
`create_date` datetime DEFAULT NULL COMMENT '创建时间',
`update_date` datetime DEFAULT NULL COMMENT '修改时间',
`store_id` int(11) DEFAULT '0' COMMENT '商家编号',
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_notice
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_order_action`
-- ----------------------------
DROP TABLE IF EXISTS `hai_order_action`;
CREATE TABLE `hai_order_action` (
`action_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`order_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`action_user` varchar(30) NOT NULL DEFAULT '',
`order_status` tinyint(1) unsigned NOT NULL DEFAULT '0',
`shipping_status` tinyint(1) unsigned NOT NULL DEFAULT '0',
`pay_status` tinyint(1) unsigned NOT NULL DEFAULT '0',
`action_place` tinyint(1) unsigned NOT NULL DEFAULT '0',
`action_note` varchar(255) NOT NULL DEFAULT '',
`log_time` int(11) unsigned NOT NULL DEFAULT '0',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`action_id`),
KEY `order_id` (`order_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_order_action
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_order_goods`
-- ----------------------------
DROP TABLE IF EXISTS `hai_order_goods`;
CREATE TABLE `hai_order_goods` (
`rec_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`order_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`sub_order_sn` varchar(50) DEFAULT NULL COMMENT '商家子订单号',
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_name` varchar(120) NOT NULL DEFAULT '',
`goods_sn` varchar(60) NOT NULL DEFAULT '',
`product_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_number` smallint(5) unsigned NOT NULL DEFAULT '1',
`market_price` decimal(10,2) NOT NULL DEFAULT '0.00',
`goods_price` decimal(10,2) NOT NULL DEFAULT '0.00',
`goods_attr` text NOT NULL,
`goods_thumb` varchar(255) DEFAULT NULL,
`send_number` smallint(5) unsigned NOT NULL DEFAULT '0',
`is_real` tinyint(1) unsigned NOT NULL DEFAULT '0',
`extension_code` varchar(30) NOT NULL DEFAULT '',
`parent_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`is_gift` smallint(5) unsigned NOT NULL DEFAULT '0',
`goods_attr_id` varchar(255) NOT NULL DEFAULT '',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`rec_id`),
KEY `order_id` (`order_id`),
KEY `goods_id` (`goods_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_order_goods
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_order_info`
-- ----------------------------
DROP TABLE IF EXISTS `hai_order_info`;
CREATE TABLE `hai_order_info` (
`order_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`order_sn` varchar(30) NOT NULL DEFAULT '',
`user_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`order_status` tinyint(1) unsigned NOT NULL DEFAULT '0',
`shipping_status` tinyint(1) unsigned NOT NULL DEFAULT '0',
`pay_status` tinyint(1) unsigned NOT NULL DEFAULT '0',
`consignee` varchar(60) NOT NULL DEFAULT '',
`country` smallint(5) unsigned NOT NULL DEFAULT '0',
`province` smallint(5) unsigned NOT NULL DEFAULT '0',
`city` smallint(5) unsigned NOT NULL DEFAULT '0',
`county` smallint(5) NOT NULL DEFAULT '0',
`district` smallint(5) unsigned DEFAULT '0',
`street` smallint(5) DEFAULT '0',
`address` varchar(255) NOT NULL DEFAULT '',
`zipcode` varchar(60) NOT NULL DEFAULT '',
`tel` varchar(60) NOT NULL DEFAULT '',
`mobile` varchar(60) NOT NULL DEFAULT '',
`email` varchar(60) NOT NULL DEFAULT '',
`best_time` varchar(120) NOT NULL DEFAULT '',
`sign_building` varchar(120) NOT NULL DEFAULT '',
`postscript` varchar(255) NOT NULL DEFAULT '',
`shipping_id` tinyint(3) NOT NULL DEFAULT '0',
`shipping_name` varchar(120) NOT NULL DEFAULT '',
`pay_id` tinyint(3) NOT NULL DEFAULT '0',
`pay_name` varchar(120) NOT NULL DEFAULT '',
`how_oos` varchar(120) NOT NULL DEFAULT '',
`how_surplus` varchar(120) NOT NULL DEFAULT '',
`pack_name` varchar(120) NOT NULL DEFAULT '',
`card_name` varchar(120) NOT NULL DEFAULT '',
`card_message` varchar(255) NOT NULL DEFAULT '',
`inv_payee` varchar(120) NOT NULL DEFAULT '',
`inv_content` varchar(120) NOT NULL DEFAULT '',
`goods_amount` decimal(10,2) NOT NULL DEFAULT '0.00',
`shipping_fee` decimal(10,2) NOT NULL DEFAULT '0.00',
`insure_fee` decimal(10,2) NOT NULL DEFAULT '0.00',
`pay_fee` decimal(10,2) NOT NULL DEFAULT '0.00',
`pack_fee` decimal(10,2) NOT NULL DEFAULT '0.00',
`card_fee` decimal(10,2) NOT NULL DEFAULT '0.00',
`money_paid` decimal(10,2) NOT NULL DEFAULT '0.00',
`surplus` decimal(10,2) NOT NULL DEFAULT '0.00',
`integral` int(10) unsigned NOT NULL DEFAULT '0',
`integral_money` decimal(10,2) NOT NULL DEFAULT '0.00',
`bonus` decimal(10,2) NOT NULL DEFAULT '0.00',
`order_amount` decimal(10,2) NOT NULL DEFAULT '0.00',
`from_ad` smallint(5) NOT NULL DEFAULT '0',
`referer` varchar(255) NOT NULL DEFAULT '',
`add_time` int(10) unsigned NOT NULL DEFAULT '0',
`confirm_time` int(10) unsigned NOT NULL DEFAULT '0',
`pay_time` int(10) unsigned NOT NULL DEFAULT '0',
`shipping_time` int(10) unsigned NOT NULL DEFAULT '0',
`pack_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
`card_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
`bonus_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`invoice_no` varchar(255) NOT NULL DEFAULT '',
`extension_code` varchar(30) NOT NULL DEFAULT '',
`extension_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`to_buyer` varchar(255) NOT NULL DEFAULT '',
`pay_note` varchar(255) NOT NULL DEFAULT '',
`agency_id` smallint(5) unsigned NOT NULL,
`inv_type` varchar(60) NOT NULL,
`tax` decimal(10,2) NOT NULL,
`is_separate` tinyint(1) NOT NULL DEFAULT '0',
`parent_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`discount` decimal(10,2) NOT NULL,
`store_id` int(11) DEFAULT NULL,
`remark` text COMMENT '备注',
`is_void` enum('1','0') DEFAULT '1',
PRIMARY KEY (`order_id`),
UNIQUE KEY `order_sn` (`order_sn`),
KEY `user_id` (`user_id`),
KEY `order_status` (`order_status`),
KEY `shipping_status` (`shipping_status`),
KEY `pay_status` (`pay_status`),
KEY `shipping_id` (`shipping_id`),
KEY `pay_id` (`pay_id`),
KEY `extension_code` (`extension_code`,`extension_id`),
KEY `agency_id` (`agency_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_order_info
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_order_pay_extends`
-- ----------------------------
DROP TABLE IF EXISTS `hai_order_pay_extends`;
CREATE TABLE `hai_order_pay_extends` (
`order_pay_extends_id` bigint(20) NOT NULL AUTO_INCREMENT,
`order_pay_id` bigint(20) DEFAULT NULL,
`realname` varchar(50) DEFAULT NULL,
`mobile` varchar(20) DEFAULT NULL,
`openid` varchar(100) DEFAULT NULL,
`face` varchar(200) DEFAULT NULL COMMENT '头像',
`order_body` text,
`prepay_id` varchar(100) DEFAULT NULL,
`guestbook` varchar(200) DEFAULT NULL COMMENT '留言',
`createdate` datetime DEFAULT NULL,
`remark` text,
`anonymous` int(5) DEFAULT '0' COMMENT '0:非匿名,1:匿名,不显示名称',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`order_pay_extends_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_order_pay_extends
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_order_pay_record`
-- ----------------------------
DROP TABLE IF EXISTS `hai_order_pay_record`;
CREATE TABLE `hai_order_pay_record` (
`order_pay_id` bigint(20) NOT NULL AUTO_INCREMENT,
`wxid` int(11) DEFAULT NULL,
`order_sn` varchar(100) DEFAULT NULL COMMENT '支付订单编号',
`order_pay_source` enum('unionpay','alipay','paybill','weixin') DEFAULT NULL,
`order_status` int(5) DEFAULT NULL COMMENT '0:刚插入,1:支付成功,2:取消支付,3:支付失败',
`amount` int(11) DEFAULT NULL,
`table_name` varchar(50) DEFAULT NULL COMMENT '对应记录表',
`table_id` bigint(20) DEFAULT NULL COMMENT '对应表格的编号',
`store_id` int(11) DEFAULT NULL,
`user_id` bigint(20) DEFAULT NULL,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
`remark` text COMMENT '备注',
`order_body` text,
`prepay_id` varchar(100) DEFAULT NULL,
PRIMARY KEY (`order_pay_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COMMENT='订单支付记录,包括微信,支付宝,银联等多种支付\r\n';
-- ----------------------------
-- Records of hai_order_pay_record
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_pack`
-- ----------------------------
DROP TABLE IF EXISTS `hai_pack`;
CREATE TABLE `hai_pack` (
`pack_id` tinyint(3) unsigned NOT NULL AUTO_INCREMENT,
`pack_name` varchar(120) NOT NULL DEFAULT '',
`pack_img` varchar(255) NOT NULL DEFAULT '',
`pack_fee` decimal(6,2) unsigned NOT NULL DEFAULT '0.00',
`free_money` smallint(5) unsigned NOT NULL DEFAULT '0',
`pack_desc` varchar(255) NOT NULL DEFAULT '',
PRIMARY KEY (`pack_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_pack
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_package_goods`
-- ----------------------------
DROP TABLE IF EXISTS `hai_package_goods`;
CREATE TABLE `hai_package_goods` (
`package_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`product_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_number` smallint(5) unsigned NOT NULL DEFAULT '1',
`admin_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`package_id`,`goods_id`,`admin_id`,`product_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_package_goods
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_payment`
-- ----------------------------
DROP TABLE IF EXISTS `hai_payment`;
CREATE TABLE `hai_payment` (
`pay_id` tinyint(3) unsigned NOT NULL AUTO_INCREMENT,
`pay_code` varchar(20) NOT NULL DEFAULT '',
`pay_name` varchar(120) NOT NULL DEFAULT '',
`pay_fee` varchar(10) NOT NULL DEFAULT '0',
`pay_desc` text NOT NULL,
`pay_order` tinyint(3) unsigned NOT NULL DEFAULT '0',
`pay_config` text NOT NULL,
`enabled` tinyint(1) unsigned NOT NULL DEFAULT '1',
`is_default` tinyint(1) NOT NULL DEFAULT '0',
`is_cod` tinyint(1) unsigned NOT NULL DEFAULT '0',
`is_online` tinyint(1) unsigned NOT NULL DEFAULT '0',
`className` varchar(20) DEFAULT NULL COMMENT '样式名称',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`pay_id`),
UNIQUE KEY `pay_code` (`pay_code`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_payment
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_pay_log`
-- ----------------------------
DROP TABLE IF EXISTS `hai_pay_log`;
CREATE TABLE `hai_pay_log` (
`log_id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`order_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`order_amount` decimal(10,2) unsigned NOT NULL,
`order_type` tinyint(1) unsigned NOT NULL DEFAULT '0',
`is_paid` tinyint(1) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`log_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_pay_log
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_plugins`
-- ----------------------------
DROP TABLE IF EXISTS `hai_plugins`;
CREATE TABLE `hai_plugins` (
`code` varchar(30) NOT NULL DEFAULT '',
`version` varchar(10) NOT NULL DEFAULT '',
`library` varchar(255) NOT NULL DEFAULT '',
`assign` tinyint(1) unsigned NOT NULL DEFAULT '0',
`install_date` int(10) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`code`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_plugins
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_products`
-- ----------------------------
DROP TABLE IF EXISTS `hai_products`;
CREATE TABLE `hai_products` (
`product_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_attr` varchar(50) DEFAULT NULL,
`product_sn` varchar(60) DEFAULT NULL,
`product_number` smallint(5) unsigned DEFAULT '0',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`product_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_products
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_region`
-- ----------------------------
DROP TABLE IF EXISTS `hai_region`;
CREATE TABLE `hai_region` (
`region_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`parent_id` smallint(5) unsigned NOT NULL DEFAULT '0',
`region_name` varchar(120) NOT NULL DEFAULT '',
`region_type` tinyint(1) NOT NULL DEFAULT '2',
`agency_id` smallint(5) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`region_id`),
KEY `parent_id` (`parent_id`),
KEY `region_type` (`region_type`),
KEY `agency_id` (`agency_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_region
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_reg_extend_info`
-- ----------------------------
DROP TABLE IF EXISTS `hai_reg_extend_info`;
CREATE TABLE `hai_reg_extend_info` (
`Id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`user_id` mediumint(8) unsigned NOT NULL,
`reg_field_id` int(10) unsigned NOT NULL,
`reg_field_name` varchar(60) DEFAULT NULL,
`content` text NOT NULL,
PRIMARY KEY (`Id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_reg_extend_info
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_reg_fields`
-- ----------------------------
DROP TABLE IF EXISTS `hai_reg_fields`;
CREATE TABLE `hai_reg_fields` (
`id` tinyint(3) unsigned NOT NULL AUTO_INCREMENT,
`reg_field_name` varchar(60) NOT NULL,
`reg_field_cn_name` varchar(200) DEFAULT NULL,
`dis_order` tinyint(3) unsigned NOT NULL DEFAULT '100',
`display` tinyint(1) unsigned NOT NULL DEFAULT '1',
`type` tinyint(1) unsigned NOT NULL DEFAULT '0',
`is_need` tinyint(1) unsigned NOT NULL DEFAULT '1',
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_reg_fields
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_role`
-- ----------------------------
DROP TABLE IF EXISTS `hai_role`;
CREATE TABLE `hai_role` (
`role_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`role_name` varchar(60) NOT NULL DEFAULT '',
`action_list` text NOT NULL,
`role_describe` text,
PRIMARY KEY (`role_id`),
KEY `user_name` (`role_name`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_role
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_searchengine`
-- ----------------------------
DROP TABLE IF EXISTS `hai_searchengine`;
CREATE TABLE `hai_searchengine` (
`date` date NOT NULL DEFAULT '0000-00-00',
`searchengine` varchar(20) NOT NULL DEFAULT '',
`count` mediumint(8) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`date`,`searchengine`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_searchengine
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_sectors`
-- ----------------------------
DROP TABLE IF EXISTS `hai_sectors`;
CREATE TABLE `hai_sectors` (
`sectors_id` int(11) unsigned NOT NULL AUTO_INCREMENT,
`sectors_name` varchar(50) DEFAULT NULL,
`parent_id` int(11) DEFAULT NULL,
`store_id` int(11) DEFAULT NULL,
`is_top` smallint(1) DEFAULT NULL COMMENT '是否热门榜单',
`order` int(11) DEFAULT '1' COMMENT '排序',
`is_header` smallint(1) DEFAULT NULL COMMENT 'parent_id为0时,此值为1就是显示在header上面',
PRIMARY KEY (`sectors_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_sectors
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_sessions`
-- ----------------------------
DROP TABLE IF EXISTS `hai_sessions`;
CREATE TABLE `hai_sessions` (
`sesskey` char(32) CHARACTER SET utf8 COLLATE utf8_bin NOT NULL DEFAULT '',
`expiry` int(10) unsigned NOT NULL DEFAULT '0',
`userid` mediumint(8) unsigned NOT NULL DEFAULT '0',
`adminid` mediumint(8) unsigned NOT NULL DEFAULT '0',
`ip` char(15) NOT NULL DEFAULT '',
`user_name` varchar(60) NOT NULL,
`user_rank` tinyint(3) NOT NULL,
`discount` decimal(3,2) NOT NULL,
`email` varchar(60) NOT NULL,
`data` char(255) NOT NULL DEFAULT '',
PRIMARY KEY (`sesskey`),
KEY `expiry` (`expiry`)
) ENGINE=MEMORY DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_sessions
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_sessions_data`
-- ----------------------------
DROP TABLE IF EXISTS `hai_sessions_data`;
CREATE TABLE `hai_sessions_data` (
`sesskey` varchar(32) CHARACTER SET utf8 COLLATE utf8_bin NOT NULL DEFAULT '',
`expiry` int(10) unsigned NOT NULL DEFAULT '0',
`data` longtext NOT NULL,
PRIMARY KEY (`sesskey`),
KEY `expiry` (`expiry`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_sessions_data
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_shipping`
-- ----------------------------
DROP TABLE IF EXISTS `hai_shipping`;
CREATE TABLE `hai_shipping` (
`shipping_id` tinyint(3) unsigned NOT NULL AUTO_INCREMENT,
`shipping_code` varchar(20) NOT NULL DEFAULT '',
`shipping_name` varchar(120) NOT NULL DEFAULT '',
`shipping_desc` varchar(255) NOT NULL DEFAULT '',
`insure` varchar(10) NOT NULL DEFAULT '0',
`support_cod` tinyint(1) unsigned NOT NULL DEFAULT '0',
`enabled` tinyint(1) unsigned NOT NULL DEFAULT '0',
`is_default` tinyint(1) DEFAULT '0',
`shipping_print` text NOT NULL,
`print_bg` varchar(255) DEFAULT NULL,
`config_lable` text,
`print_model` tinyint(1) DEFAULT '0',
`shipping_order` tinyint(3) unsigned NOT NULL DEFAULT '0',
`store_id` int(11) DEFAULT NULL,
`className` varchar(20) DEFAULT NULL,
PRIMARY KEY (`shipping_id`),
KEY `shipping_code` (`shipping_code`,`enabled`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 CHECKSUM=1 DELAY_KEY_WRITE=1 ROW_FORMAT=DYNAMIC;
-- ----------------------------
-- Records of hai_shipping
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_shipping_area`
-- ----------------------------
DROP TABLE IF EXISTS `hai_shipping_area`;
CREATE TABLE `hai_shipping_area` (
`shipping_area_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`shipping_area_name` varchar(150) NOT NULL DEFAULT '',
`shipping_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
`configure` text NOT NULL,
PRIMARY KEY (`shipping_area_id`),
KEY `shipping_id` (`shipping_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_shipping_area
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_shop_config`
-- ----------------------------
DROP TABLE IF EXISTS `hai_shop_config`;
CREATE TABLE `hai_shop_config` (
`id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`parent_id` smallint(5) unsigned NOT NULL DEFAULT '0',
`code` varchar(30) NOT NULL DEFAULT '',
`code_text` varchar(20) DEFAULT NULL,
`type` varchar(10) NOT NULL DEFAULT '',
`store_range` varchar(255) NOT NULL DEFAULT '',
`store_dir` varchar(255) NOT NULL DEFAULT '',
`value` text NOT NULL,
`sort_order` tinyint(3) unsigned NOT NULL DEFAULT '1',
`store_id` int(11) DEFAULT NULL,
`isvoid` enum('0','1') DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_shop_config
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_snatch_log`
-- ----------------------------
DROP TABLE IF EXISTS `hai_snatch_log`;
CREATE TABLE `hai_snatch_log` (
`log_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`snatch_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
`user_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`bid_price` decimal(10,2) NOT NULL DEFAULT '0.00',
`bid_time` int(10) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`log_id`),
KEY `snatch_id` (`snatch_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_snatch_log
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_stats`
-- ----------------------------
DROP TABLE IF EXISTS `hai_stats`;
CREATE TABLE `hai_stats` (
`access_time` int(10) unsigned NOT NULL DEFAULT '0',
`ip_address` varchar(15) NOT NULL DEFAULT '',
`visit_times` smallint(5) unsigned NOT NULL DEFAULT '1',
`browser` varchar(60) NOT NULL DEFAULT '',
`system` varchar(20) NOT NULL DEFAULT '',
`language` varchar(20) NOT NULL DEFAULT '',
`area` varchar(30) NOT NULL DEFAULT '',
`referer_domain` varchar(100) NOT NULL DEFAULT '',
`referer_path` varchar(200) NOT NULL DEFAULT '',
`access_url` varchar(255) NOT NULL DEFAULT '',
`store_id` int(11) DEFAULT NULL,
KEY `access_time` (`access_time`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_stats
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_store`
-- ----------------------------
DROP TABLE IF EXISTS `hai_store`;
CREATE TABLE `hai_store` (
`store_id` int(10) unsigned NOT NULL DEFAULT '0',
`store_name` varchar(100) NOT NULL DEFAULT '',
`owner_name` varchar(60) NOT NULL DEFAULT '',
`owner_card` varchar(60) NOT NULL DEFAULT '',
`region_id` int(10) unsigned DEFAULT NULL,
`region_name` varchar(100) DEFAULT NULL,
`address` varchar(255) NOT NULL DEFAULT '',
`zipcode` varchar(20) NOT NULL DEFAULT '',
`tel` varchar(60) NOT NULL DEFAULT '',
`sgrade` tinyint(3) unsigned NOT NULL DEFAULT '0',
`apply_remark` varchar(255) NOT NULL DEFAULT '',
`credit_value` int(10) NOT NULL DEFAULT '0',
`praise_rate` decimal(5,2) unsigned NOT NULL DEFAULT '0.00',
`domain` varchar(60) DEFAULT NULL,
`state` tinyint(3) unsigned NOT NULL DEFAULT '0',
`close_reason` varchar(255) NOT NULL DEFAULT '',
`add_time` int(10) unsigned DEFAULT NULL,
`end_time` int(10) unsigned NOT NULL DEFAULT '0',
`certification` varchar(255) DEFAULT NULL,
`sort_order` smallint(5) unsigned NOT NULL DEFAULT '0',
`recommended` tinyint(4) NOT NULL DEFAULT '0',
`theme` varchar(60) NOT NULL DEFAULT '',
`store_banner` varchar(255) DEFAULT NULL,
`store_logo` varchar(255) DEFAULT NULL,
`description` text,
`image_1` varchar(255) NOT NULL DEFAULT '',
`image_2` varchar(255) NOT NULL DEFAULT '',
`image_3` varchar(255) NOT NULL DEFAULT '',
`im_qq` varchar(60) NOT NULL DEFAULT '',
`im_ww` varchar(60) NOT NULL DEFAULT '',
`im_msn` varchar(60) NOT NULL DEFAULT '',
`enable_groupbuy` tinyint(1) unsigned NOT NULL DEFAULT '0',
`enable_radar` tinyint(1) unsigned NOT NULL DEFAULT '1',
PRIMARY KEY (`store_id`),
KEY `store_name` (`store_name`),
KEY `owner_name` (`owner_name`),
KEY `region_id` (`region_id`),
KEY `domain` (`domain`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_store
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_store_appkey_secret`
-- ----------------------------
DROP TABLE IF EXISTS `hai_store_appkey_secret`;
CREATE TABLE `hai_store_appkey_secret` (
`app_id` int(5) NOT NULL AUTO_INCREMENT,
`appkey` varchar(64) NOT NULL,
`secret` varchar(128) NOT NULL,
`store_id` int(11) NOT NULL,
`logo` varchar(200) DEFAULT NULL,
`title` varchar(100) DEFAULT NULL,
`desc` longtext,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
`isvalid` enum('0','1') NOT NULL DEFAULT '1',
PRIMARY KEY (`app_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 ROW_FORMAT=COMPACT;
-- ----------------------------
-- Records of hai_store_appkey_secret
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_suppliers`
-- ----------------------------
DROP TABLE IF EXISTS `hai_suppliers`;
CREATE TABLE `hai_suppliers` (
`suppliers_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`suppliers_name` varchar(255) DEFAULT NULL,
`suppliers_desc` mediumtext,
`is_check` tinyint(1) unsigned NOT NULL DEFAULT '1',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`suppliers_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_suppliers
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_tag`
-- ----------------------------
DROP TABLE IF EXISTS `hai_tag`;
CREATE TABLE `hai_tag` (
`tag_id` mediumint(8) NOT NULL AUTO_INCREMENT,
`user_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`tag_words` varchar(255) NOT NULL DEFAULT '',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`tag_id`),
KEY `user_id` (`user_id`),
KEY `goods_id` (`goods_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_tag
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_taobao`
-- ----------------------------
DROP TABLE IF EXISTS `hai_taobao`;
CREATE TABLE `hai_taobao` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`session` varchar(255) DEFAULT NULL,
`nick` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_taobao
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_taobao_order`
-- ----------------------------
DROP TABLE IF EXISTS `hai_taobao_order`;
CREATE TABLE `hai_taobao_order` (
`order_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`order_sn` varchar(255) NOT NULL DEFAULT '',
`order_title` varchar(255) NOT NULL DEFAULT '0',
`order_status` varchar(255) NOT NULL DEFAULT '0',
`shipping_status` tinyint(1) unsigned NOT NULL DEFAULT '0',
`pay_status` tinyint(1) unsigned NOT NULL DEFAULT '0',
`consignee` varchar(60) NOT NULL DEFAULT '',
`country` smallint(5) unsigned NOT NULL DEFAULT '0',
`province` smallint(5) unsigned NOT NULL DEFAULT '0',
`city` smallint(5) unsigned NOT NULL DEFAULT '0',
`district` smallint(5) unsigned NOT NULL DEFAULT '0',
`address` varchar(255) NOT NULL DEFAULT '',
`zipcode` varchar(60) NOT NULL DEFAULT '',
`tel` varchar(60) NOT NULL DEFAULT '',
`mobile` varchar(60) NOT NULL DEFAULT '',
`email` varchar(60) NOT NULL DEFAULT '',
`best_time` varchar(120) NOT NULL DEFAULT '',
`sign_building` varchar(120) NOT NULL DEFAULT '',
`postscript` varchar(255) NOT NULL DEFAULT '',
`shipping_id` tinyint(3) NOT NULL DEFAULT '0',
`shipping_name` varchar(120) NOT NULL DEFAULT '',
`pay_id` tinyint(3) NOT NULL DEFAULT '0',
`pay_name` varchar(120) NOT NULL DEFAULT '',
`how_oos` varchar(120) NOT NULL DEFAULT '',
`how_surplus` varchar(120) NOT NULL DEFAULT '',
`pack_name` varchar(120) NOT NULL DEFAULT '',
`card_name` varchar(120) NOT NULL DEFAULT '',
`card_message` varchar(255) NOT NULL DEFAULT '',
`inv_payee` varchar(120) NOT NULL DEFAULT '',
`inv_content` varchar(120) NOT NULL DEFAULT '',
`goods_amount` decimal(10,2) NOT NULL DEFAULT '0.00',
`shipping_fee` decimal(10,2) NOT NULL DEFAULT '0.00',
`insure_fee` decimal(10,2) NOT NULL DEFAULT '0.00',
`pay_fee` decimal(10,2) NOT NULL DEFAULT '0.00',
`pack_fee` decimal(10,2) NOT NULL DEFAULT '0.00',
`card_fee` decimal(10,2) NOT NULL DEFAULT '0.00',
`money_paid` decimal(10,2) NOT NULL DEFAULT '0.00',
`surplus` decimal(10,2) NOT NULL DEFAULT '0.00',
`integral` int(10) unsigned NOT NULL DEFAULT '0',
`integral_money` decimal(10,2) NOT NULL DEFAULT '0.00',
`bonus` decimal(10,2) NOT NULL DEFAULT '0.00',
`order_amount` decimal(10,2) NOT NULL DEFAULT '0.00',
`from_ad` smallint(5) NOT NULL DEFAULT '0',
`referer` varchar(255) NOT NULL DEFAULT '',
`add_time` datetime NOT NULL DEFAULT '0000-00-00 00:00:00',
`confirm_time` int(10) unsigned NOT NULL DEFAULT '0',
`pay_time` datetime NOT NULL DEFAULT '0000-00-00 00:00:00',
`shipping_time` datetime NOT NULL DEFAULT '0000-00-00 00:00:00',
`pack_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
`card_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
`bonus_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`invoice_no` varchar(255) NOT NULL DEFAULT '',
`extension_code` varchar(30) NOT NULL DEFAULT '',
`extension_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`to_buyer` varchar(255) NOT NULL DEFAULT '',
`pay_note` varchar(255) NOT NULL DEFAULT '',
`agency_id` smallint(5) unsigned NOT NULL,
`inv_type` varchar(60) NOT NULL,
`tax` decimal(10,2) NOT NULL,
`is_separate` tinyint(1) NOT NULL DEFAULT '0',
`parent_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`discount` decimal(10,2) NOT NULL,
`adjust_fee` decimal(10,2) DEFAULT NULL,
`buyer_rate` varchar(6) DEFAULT NULL,
`discount_fee` decimal(10,2) DEFAULT NULL,
`order_iid` varchar(40) DEFAULT NULL,
`goods_price` decimal(10,2) DEFAULT NULL,
`refund_status` varchar(10) DEFAULT NULL,
`seller_rate` varchar(6) DEFAULT NULL,
`seller_type` varchar(5) DEFAULT NULL,
`buyer_nick` varchar(255) DEFAULT NULL,
`goods_num` int(4) DEFAULT NULL,
`user_name` varchar(10) DEFAULT NULL,
`chick_status` varchar(40) DEFAULT NULL,
PRIMARY KEY (`order_id`),
UNIQUE KEY `order_sn` (`order_sn`),
KEY `user_id` (`order_title`),
KEY `order_status` (`order_status`),
KEY `shipping_status` (`shipping_status`),
KEY `pay_status` (`pay_status`),
KEY `shipping_id` (`shipping_id`),
KEY `pay_id` (`pay_id`),
KEY `extension_code` (`extension_code`,`extension_id`),
KEY `agency_id` (`agency_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_taobao_order
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_template`
-- ----------------------------
DROP TABLE IF EXISTS `hai_template`;
CREATE TABLE `hai_template` (
`filename` varchar(30) NOT NULL DEFAULT '',
`region` varchar(40) NOT NULL DEFAULT '',
`library` varchar(40) NOT NULL DEFAULT '',
`sort_order` tinyint(1) unsigned NOT NULL DEFAULT '0',
`id` smallint(5) unsigned NOT NULL DEFAULT '0',
`number` tinyint(1) unsigned NOT NULL DEFAULT '5',
`type` tinyint(1) unsigned NOT NULL DEFAULT '0',
`theme` varchar(60) NOT NULL DEFAULT '',
`remarks` varchar(30) NOT NULL DEFAULT '',
KEY `filename` (`filename`,`region`),
KEY `theme` (`theme`),
KEY `remarks` (`remarks`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_template
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_time_axisn`
-- ----------------------------
DROP TABLE IF EXISTS `hai_time_axisn`;
CREATE TABLE `hai_time_axisn` (
`time_id` int(11) NOT NULL AUTO_INCREMENT,
`title` varchar(100) DEFAULT NULL,
`summary` varchar(200) DEFAULT NULL,
`content` text,
`his_date` date DEFAULT NULL,
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`time_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_time_axisn
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_topic`
-- ----------------------------
DROP TABLE IF EXISTS `hai_topic`;
CREATE TABLE `hai_topic` (
`topic_id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`title` varchar(255) NOT NULL DEFAULT '''''',
`intro` text NOT NULL,
`start_time` int(11) NOT NULL DEFAULT '0',
`end_time` int(10) NOT NULL DEFAULT '0',
`data` text NOT NULL,
`template` varchar(255) NOT NULL DEFAULT '''''',
`css` text NOT NULL,
`topic_img` varchar(255) DEFAULT NULL,
`title_pic` varchar(255) DEFAULT NULL,
`base_style` char(6) DEFAULT NULL,
`htmls` mediumtext,
`keywords` varchar(255) DEFAULT NULL,
`description` varchar(255) DEFAULT NULL,
KEY `topic_id` (`topic_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_topic
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_translation_info`
-- ----------------------------
DROP TABLE IF EXISTS `hai_translation_info`;
CREATE TABLE `hai_translation_info` (
`t_id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(10) DEFAULT NULL,
`Translation_name` varchar(100) DEFAULT NULL,
`age` int(4) DEFAULT NULL,
`sex` int(2) DEFAULT NULL,
`face_path` varchar(200) DEFAULT NULL COMMENT '头像图片',
`xueli` varchar(50) DEFAULT NULL COMMENT '学历',
`school` varchar(100) DEFAULT NULL,
`translation_type` varchar(200) DEFAULT NULL COMMENT '翻译类型',
`city` varchar(50) DEFAULT NULL,
`xinshui` varchar(50) DEFAULT NULL,
`self_introduction` text,
`experience` text,
`telephone` varchar(50) DEFAULT NULL,
`mobilephone` varchar(50) DEFAULT NULL,
`qq` varchar(50) DEFAULT NULL,
`weixin` varchar(50) DEFAULT NULL,
`email` varchar(50) DEFAULT NULL,
`address` varchar(200) DEFAULT NULL,
`pass` smallint(1) DEFAULT '0' COMMENT '是否通过审核',
`store_id` int(11) DEFAULT NULL,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
PRIMARY KEY (`t_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_translation_info
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_translation_job`
-- ----------------------------
DROP TABLE IF EXISTS `hai_translation_job`;
CREATE TABLE `hai_translation_job` (
`t_job_id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) DEFAULT NULL,
`title` varchar(200) DEFAULT NULL COMMENT '标题',
`issue_date` date DEFAULT NULL COMMENT '发布日期',
`end_date` date DEFAULT NULL COMMENT '截止日期',
`city` varchar(50) DEFAULT NULL COMMENT '所在城市',
`job_type` varchar(100) DEFAULT NULL COMMENT '工作类型',
`xinshui` varchar(50) DEFAULT NULL COMMENT '薪水',
`quantity` varchar(50) DEFAULT NULL COMMENT '数量',
`sex` int(1) DEFAULT NULL COMMENT '性别',
`height` varchar(50) DEFAULT NULL COMMENT '身高',
`linkman` varchar(50) DEFAULT NULL COMMENT '联系人',
`mobilephone` varchar(50) DEFAULT NULL COMMENT '手机',
`QQ` varchar(50) DEFAULT NULL COMMENT 'QQ',
`email` varchar(50) DEFAULT NULL COMMENT '邮箱',
`job_yao_qiu` text COMMENT '招聘要求',
`pass` smallint(1) DEFAULT '0' COMMENT '是否通过审核',
`store_id` int(10) DEFAULT NULL,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
PRIMARY KEY (`t_job_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 ROW_FORMAT=COMPACT;
-- ----------------------------
-- Records of hai_translation_job
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_users`
-- ----------------------------
DROP TABLE IF EXISTS `hai_users`;
CREATE TABLE `hai_users` (
`user_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`email` varchar(100) DEFAULT NULL,
`user_name` varchar(100) DEFAULT NULL,
`password` varchar(64) DEFAULT NULL,
`nickname` varchar(100) DEFAULT NULL COMMENT '昵称',
`realname` varchar(100) DEFAULT NULL COMMENT '真实姓名',
`question` varchar(255) DEFAULT NULL,
`answer` varchar(255) DEFAULT '',
`sex` tinyint(1) unsigned DEFAULT '0',
`birthday` date DEFAULT '0000-00-00',
`user_money` decimal(10,2) DEFAULT '0.00',
`frozen_money` decimal(10,2) DEFAULT '0.00',
`pay_points` int(10) unsigned DEFAULT '0',
`rank_points` int(10) unsigned DEFAULT '0',
`address` varchar(200) DEFAULT NULL,
`address_id` mediumint(8) unsigned DEFAULT '0',
`reg_time` datetime DEFAULT NULL,
`last_login` datetime DEFAULT NULL,
`last_time` datetime DEFAULT '0000-00-00 00:00:00',
`last_ip` varchar(15) DEFAULT '',
`visit_count` smallint(5) unsigned DEFAULT '0',
`user_rank` tinyint(3) unsigned DEFAULT '0',
`is_special` tinyint(3) unsigned DEFAULT '0',
`ec_salt` varchar(10) DEFAULT NULL,
`salt` varchar(10) DEFAULT '0',
`parent_id` mediumint(9) DEFAULT '0',
`flag` tinyint(3) unsigned DEFAULT '0',
`alias` varchar(60) DEFAULT NULL,
`msn` varchar(60) DEFAULT NULL,
`qq` varchar(20) DEFAULT NULL,
`office_phone` varchar(20) DEFAULT NULL,
`home_phone` varchar(20) DEFAULT NULL,
`mobile_phone` varchar(20) DEFAULT NULL,
`is_validated` tinyint(3) unsigned DEFAULT '0',
`credit_line` decimal(10,2) unsigned DEFAULT NULL,
`passwd_question` varchar(50) DEFAULT NULL,
`passwd_answer` varchar(255) DEFAULT NULL,
`store_id` int(11) DEFAULT NULL,
`country` smallint(6) DEFAULT NULL,
`province` smallint(6) DEFAULT NULL,
`city` smallint(6) DEFAULT NULL,
`county` smallint(6) DEFAULT NULL,
`district` smallint(6) DEFAULT NULL,
`street` smallint(6) DEFAULT NULL,
`self_description` text COMMENT '自我描述',
`user_type` smallint(1) DEFAULT '0' COMMENT '0:普通用户,1:企业',
`face_image` varchar(200) DEFAULT NULL COMMENT '头像',
`openid` varchar(100) DEFAULT NULL,
`subscribe` int(5) DEFAULT NULL,
PRIMARY KEY (`user_id`),
UNIQUE KEY `user_name` (`user_name`),
KEY `email` (`email`),
KEY `parent_id` (`parent_id`),
KEY `flag` (`flag`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_users
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_user_account`
-- ----------------------------
DROP TABLE IF EXISTS `hai_user_account`;
CREATE TABLE `hai_user_account` (
`id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`user_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`admin_user` varchar(255) NOT NULL,
`amount` decimal(10,2) NOT NULL,
`add_time` int(10) NOT NULL DEFAULT '0',
`paid_time` int(10) NOT NULL DEFAULT '0',
`admin_note` varchar(255) NOT NULL,
`user_note` varchar(255) NOT NULL,
`process_type` tinyint(1) NOT NULL DEFAULT '0',
`payment` varchar(90) NOT NULL,
`is_paid` tinyint(1) NOT NULL DEFAULT '0',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `user_id` (`user_id`),
KEY `is_paid` (`is_paid`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_user_account
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_user_address`
-- ----------------------------
DROP TABLE IF EXISTS `hai_user_address`;
CREATE TABLE `hai_user_address` (
`address_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`address_name` varchar(50) NOT NULL DEFAULT '',
`user_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`consignee` varchar(60) NOT NULL DEFAULT '',
`email` varchar(60) NOT NULL DEFAULT '',
`country` smallint(5) NOT NULL DEFAULT '0',
`province` smallint(5) NOT NULL DEFAULT '0',
`city` smallint(5) NOT NULL DEFAULT '0',
`county` smallint(5) DEFAULT '0',
`district` smallint(5) NOT NULL DEFAULT '0',
`street` smallint(5) DEFAULT '0' COMMENT '街道办',
`address` varchar(120) NOT NULL DEFAULT '',
`zipcode` varchar(60) NOT NULL DEFAULT '',
`tel` varchar(60) NOT NULL DEFAULT '',
`mobile` varchar(60) NOT NULL DEFAULT '',
`sign_building` varchar(120) NOT NULL DEFAULT '',
`best_time` varchar(120) NOT NULL DEFAULT '',
`is_default` enum('0','1') NOT NULL DEFAULT '0' COMMENT '是否默认地址',
`store_id` int(11) DEFAULT NULL,
`update_time` datetime DEFAULT NULL COMMENT '更新日期',
`add_time` datetime DEFAULT NULL,
PRIMARY KEY (`address_id`),
KEY `user_id` (`user_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_user_address
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_user_bonus`
-- ----------------------------
DROP TABLE IF EXISTS `hai_user_bonus`;
CREATE TABLE `hai_user_bonus` (
`bonus_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`bonus_type_id` tinyint(3) unsigned NOT NULL DEFAULT '0',
`bonus_sn` bigint(20) unsigned NOT NULL DEFAULT '0',
`user_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`used_time` int(10) unsigned NOT NULL DEFAULT '0',
`order_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`emailed` tinyint(3) unsigned NOT NULL DEFAULT '0',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`bonus_id`),
KEY `user_id` (`user_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_user_bonus
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_user_feed`
-- ----------------------------
DROP TABLE IF EXISTS `hai_user_feed`;
CREATE TABLE `hai_user_feed` (
`feed_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`user_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`value_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`feed_type` tinyint(1) unsigned NOT NULL DEFAULT '0',
`is_feed` tinyint(1) unsigned NOT NULL DEFAULT '0',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`feed_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_user_feed
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_user_login`
-- ----------------------------
DROP TABLE IF EXISTS `hai_user_login`;
CREATE TABLE `hai_user_login` (
`login_id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) DEFAULT NULL,
`session_id` varchar(64) DEFAULT NULL,
`login_time` int(15) DEFAULT NULL,
`longitude` float(10,6) DEFAULT NULL,
`latitude` float(10,6) DEFAULT NULL,
`login_ip` varchar(15) DEFAULT NULL,
PRIMARY KEY (`login_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_user_login
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_user_rank`
-- ----------------------------
DROP TABLE IF EXISTS `hai_user_rank`;
CREATE TABLE `hai_user_rank` (
`rank_id` tinyint(3) unsigned NOT NULL AUTO_INCREMENT,
`rank_name` varchar(30) NOT NULL DEFAULT '',
`min_points` int(10) unsigned NOT NULL DEFAULT '0',
`max_points` int(10) unsigned NOT NULL DEFAULT '0',
`discount` tinyint(3) unsigned NOT NULL DEFAULT '0',
`show_price` tinyint(1) unsigned NOT NULL DEFAULT '1',
`special_rank` tinyint(1) unsigned NOT NULL DEFAULT '0',
`store_id` int(11) DEFAULT NULL,
PRIMARY KEY (`rank_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_user_rank
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_virtual_card`
-- ----------------------------
DROP TABLE IF EXISTS `hai_virtual_card`;
CREATE TABLE `hai_virtual_card` (
`card_id` mediumint(8) NOT NULL AUTO_INCREMENT,
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0',
`card_sn` varchar(60) NOT NULL DEFAULT '',
`card_password` varchar(60) NOT NULL DEFAULT '',
`add_date` int(11) NOT NULL DEFAULT '0',
`end_date` int(11) NOT NULL DEFAULT '0',
`is_saled` tinyint(1) NOT NULL DEFAULT '0',
`order_sn` varchar(20) NOT NULL DEFAULT '',
`crc32` varchar(12) NOT NULL DEFAULT '0',
PRIMARY KEY (`card_id`),
KEY `goods_id` (`goods_id`),
KEY `car_sn` (`card_sn`),
KEY `is_saled` (`is_saled`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_virtual_card
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_volume_price`
-- ----------------------------
DROP TABLE IF EXISTS `hai_volume_price`;
CREATE TABLE `hai_volume_price` (
`price_type` tinyint(1) unsigned NOT NULL,
`goods_id` mediumint(8) unsigned NOT NULL,
`volume_number` smallint(5) unsigned NOT NULL DEFAULT '0',
`volume_price` decimal(10,2) NOT NULL DEFAULT '0.00',
PRIMARY KEY (`price_type`,`goods_id`,`volume_number`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_volume_price
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_vote`
-- ----------------------------
DROP TABLE IF EXISTS `hai_vote`;
CREATE TABLE `hai_vote` (
`vote_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`vote_name` varchar(250) NOT NULL DEFAULT '',
`start_time` int(11) unsigned NOT NULL DEFAULT '0',
`end_time` int(11) unsigned NOT NULL DEFAULT '0',
`can_multi` tinyint(1) unsigned NOT NULL DEFAULT '0',
`vote_count` int(10) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`vote_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_vote
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_vote_log`
-- ----------------------------
DROP TABLE IF EXISTS `hai_vote_log`;
CREATE TABLE `hai_vote_log` (
`log_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`vote_id` smallint(5) unsigned NOT NULL DEFAULT '0',
`ip_address` varchar(15) NOT NULL DEFAULT '',
`vote_time` int(10) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`log_id`),
KEY `vote_id` (`vote_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_vote_log
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_vote_option`
-- ----------------------------
DROP TABLE IF EXISTS `hai_vote_option`;
CREATE TABLE `hai_vote_option` (
`option_id` smallint(5) unsigned NOT NULL AUTO_INCREMENT,
`vote_id` smallint(5) unsigned NOT NULL DEFAULT '0',
`option_name` varchar(250) NOT NULL DEFAULT '',
`option_count` int(8) unsigned NOT NULL DEFAULT '0',
`option_order` tinyint(3) unsigned NOT NULL DEFAULT '100',
PRIMARY KEY (`option_id`),
KEY `vote_id` (`vote_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_vote_option
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_wholesale`
-- ----------------------------
DROP TABLE IF EXISTS `hai_wholesale`;
CREATE TABLE `hai_wholesale` (
`act_id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`goods_id` mediumint(8) unsigned NOT NULL,
`goods_name` varchar(255) NOT NULL,
`rank_ids` varchar(255) NOT NULL,
`prices` text NOT NULL,
`enabled` tinyint(3) unsigned NOT NULL,
PRIMARY KEY (`act_id`),
KEY `goods_id` (`goods_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_wholesale
-- ----------------------------
-- ----------------------------
-- Table structure for `mw_lbs`
-- ----------------------------
DROP TABLE IF EXISTS `mw_lbs`;
CREATE TABLE `mw_lbs` (
`mw_id` bigint(20) NOT NULL AUTO_INCREMENT,
`phone` varchar(20) DEFAULT NULL,
`other_phone` varchar(20) DEFAULT NULL,
`latitude` int(11) DEFAULT NULL,
`longitude` int(11) DEFAULT NULL,
`addtime` bigint(20) DEFAULT NULL COMMENT 'FROM_UNIXTIME( addtime, ''%Y-%m-%d %H:%i:%s'' )',
PRIMARY KEY (`mw_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of mw_lbs
-- ----------------------------
-- ----------------------------
-- Table structure for `pro_examine`
-- ----------------------------
DROP TABLE IF EXISTS `pro_examine`;
CREATE TABLE `pro_examine` (
`examine_id` int(11) NOT NULL AUTO_INCREMENT,
`examine_title` varchar(200) DEFAULT NULL,
`examine_m_content` text,
`examine_content` text,
`examine_m_result` text,
`examine_result` text,
`pro_id` int(11) DEFAULT NULL,
`plan_start_date` date DEFAULT NULL,
`plan_end_date` date DEFAULT NULL,
`truth_start_date` date DEFAULT NULL,
`truth_end_date` date DEFAULT NULL,
`user_id` int(11) DEFAULT NULL,
`dispatch_user_id` int(11) DEFAULT NULL COMMENT '指派处理的用户编号',
`progress` int(6) DEFAULT NULL,
`m_remark` text,
`remark` text,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
PRIMARY KEY (`examine_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of pro_examine
-- ----------------------------
-- ----------------------------
-- Table structure for `pro_idea`
-- ----------------------------
DROP TABLE IF EXISTS `pro_idea`;
CREATE TABLE `pro_idea` (
`idea_id` int(11) NOT NULL AUTO_INCREMENT,
`idea_title` varchar(200) DEFAULT NULL,
`idea_m_content` text,
`idea_content` text,
`idea_m_remark` text,
`idea_remark` text,
`idea_m_plan` text,
`idea_plan` text,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
`user_id` int(11) DEFAULT NULL,
`state` int(5) DEFAULT NULL,
`progress` int(3) DEFAULT NULL,
`plan_date` date DEFAULT NULL,
PRIMARY KEY (`idea_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of pro_idea
-- ----------------------------
-- ----------------------------
-- Table structure for `pro_plan`
-- ----------------------------
DROP TABLE IF EXISTS `pro_plan`;
CREATE TABLE `pro_plan` (
`plan_id` int(11) NOT NULL AUTO_INCREMENT,
`task_id` int(11) DEFAULT NULL,
`plan_title` varchar(200) DEFAULT NULL,
`plan_m_content` text,
`plan_content` text,
`plan_m_result` text,
`plan_result` text,
`pro_id` int(11) DEFAULT NULL,
`plan_start_date` date DEFAULT NULL,
`plan_end_date` date DEFAULT NULL,
`truth_start_date` date DEFAULT NULL,
`truth_end_date` date DEFAULT NULL,
`user_id` int(11) DEFAULT NULL,
`dispatch_user_id` int(11) DEFAULT NULL COMMENT '指派处理的用户编号',
`progress` int(6) DEFAULT NULL,
`m_remark` text,
`remark` text,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
PRIMARY KEY (`plan_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 ROW_FORMAT=COMPACT;
-- ----------------------------
-- Records of pro_plan
-- ----------------------------
-- ----------------------------
-- Table structure for `pro_project`
-- ----------------------------
DROP TABLE IF EXISTS `pro_project`;
CREATE TABLE `pro_project` (
`pro_id` int(11) NOT NULL AUTO_INCREMENT,
`pro_name` varchar(50) DEFAULT NULL,
`pro_data_type` int(4) DEFAULT '1' COMMENT '1:管理后台级,2:前台用户级',
`pro_admin_user_id` int(4) DEFAULT '0' COMMENT '管理员级或用户级的编号',
PRIMARY KEY (`pro_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of pro_project
-- ----------------------------
-- ----------------------------
-- Table structure for `pro_task`
-- ----------------------------
DROP TABLE IF EXISTS `pro_task`;
CREATE TABLE `pro_task` (
`task_id` int(11) NOT NULL AUTO_INCREMENT,
`task_title` varchar(200) DEFAULT NULL,
`task_m_content` text,
`task_content` text,
`task_m_result` text,
`task_result` text,
`pro_id` int(11) DEFAULT NULL,
`plan_start_date` date DEFAULT NULL,
`plan_end_date` date DEFAULT NULL,
`truth_start_date` date DEFAULT NULL,
`truth_end_date` date DEFAULT NULL,
`user_id` int(11) DEFAULT NULL,
`dispatch_user_id` int(11) DEFAULT NULL COMMENT '指派处理的用户编号',
`progress` int(6) DEFAULT NULL,
`m_remark` text,
`remark` text,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
PRIMARY KEY (`task_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 ROW_FORMAT=COMPACT;
-- ----------------------------
-- Records of pro_task
-- ----------------------------
-- ----------------------------
-- Table structure for `pro_user_project`
-- ----------------------------
DROP TABLE IF EXISTS `pro_user_project`;
CREATE TABLE `pro_user_project` (
`pro_user_id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) DEFAULT NULL,
`pro_id` int(11) DEFAULT NULL,
`state` int(5) DEFAULT '0',
PRIMARY KEY (`pro_user_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of pro_user_project
-- ----------------------------
-- ----------------------------
-- Table structure for `pro_wbs_work`
-- ----------------------------
DROP TABLE IF EXISTS `pro_wbs_work`;
CREATE TABLE `pro_wbs_work` (
`wbs_id` int(11) NOT NULL AUTO_INCREMENT,
`wbs_name` varchar(100) DEFAULT NULL,
`pro_id` int(11) DEFAULT '0',
`wbs_parent_id` int(11) DEFAULT '0',
`plan_start_date` date DEFAULT NULL,
`plan_end_date` date DEFAULT NULL,
`truth_start_date` date DEFAULT NULL,
`truth_end_date` date DEFAULT NULL,
`user_id` int(11) DEFAULT '0' COMMENT '会员编号',
`progress` int(11) DEFAULT '0' COMMENT '进度',
`sort` int(5) DEFAULT '0' COMMENT '排序',
`remark` text COMMENT '备注',
PRIMARY KEY (`wbs_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of pro_wbs_work
-- ----------------------------
-- ----------------------------
-- Table structure for `pro_work_log`
-- ----------------------------
DROP TABLE IF EXISTS `pro_work_log`;
CREATE TABLE `pro_work_log` (
`work_id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) DEFAULT NULL,
`title` varchar(200) DEFAULT NULL,
`simple_content` text,
`content` text,
`m_plan` text,
`plan` text,
`m_remark` text,
`remark` text,
`status` smallint(4) DEFAULT NULL,
`work_date` date DEFAULT NULL,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
`pro_id` int(11) DEFAULT '0',
PRIMARY KEY (`work_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of pro_work_log
-- ----------------------------
-- ----------------------------
-- Table structure for `think_access`
-- ----------------------------
DROP TABLE IF EXISTS `think_access`;
CREATE TABLE `think_access` (
`role_id` smallint(6) unsigned NOT NULL,
`node_id` smallint(6) unsigned NOT NULL,
`level` tinyint(1) NOT NULL,
`pid` smallint(6) NOT NULL,
`module` varchar(50) DEFAULT NULL,
PRIMARY KEY (`role_id`,`node_id`),
KEY `groupId` (`role_id`),
KEY `nodeId` (`node_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 COMMENT='角色对应节点的访问权限';
-- ----------------------------
-- Records of think_access
-- ----------------------------
-- ----------------------------
-- Table structure for `think_auth_group`
-- ----------------------------
DROP TABLE IF EXISTS `think_auth_group`;
CREATE TABLE `think_auth_group` (
`id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`title` char(100) NOT NULL DEFAULT '',
`status` tinyint(1) NOT NULL DEFAULT '1',
`rules` char(80) NOT NULL DEFAULT '',
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of think_auth_group
-- ----------------------------
-- ----------------------------
-- Table structure for `think_auth_group_access`
-- ----------------------------
DROP TABLE IF EXISTS `think_auth_group_access`;
CREATE TABLE `think_auth_group_access` (
`uid` mediumint(8) unsigned NOT NULL,
`group_id` mediumint(8) unsigned NOT NULL,
UNIQUE KEY `uid_group_id` (`uid`,`group_id`),
KEY `uid` (`uid`),
KEY `group_id` (`group_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of think_auth_group_access
-- ----------------------------
-- ----------------------------
-- Table structure for `think_auth_rule`
-- ----------------------------
DROP TABLE IF EXISTS `think_auth_rule`;
CREATE TABLE `think_auth_rule` (
`id` mediumint(8) unsigned NOT NULL AUTO_INCREMENT,
`name` char(80) NOT NULL DEFAULT '',
`title` char(20) NOT NULL DEFAULT '',
`status` tinyint(1) NOT NULL DEFAULT '1',
`condition` char(100) NOT NULL DEFAULT '',
PRIMARY KEY (`id`),
UNIQUE KEY `name` (`name`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of think_auth_rule
-- ----------------------------
-- ----------------------------
-- Table structure for `think_group`
-- ----------------------------
DROP TABLE IF EXISTS `think_group`;
CREATE TABLE `think_group` (
`id` smallint(3) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(25) NOT NULL,
`title` varchar(50) NOT NULL,
`create_time` datetime NOT NULL,
`update_time` datetime NOT NULL,
`status` tinyint(1) unsigned NOT NULL DEFAULT '0',
`sort` smallint(3) unsigned NOT NULL DEFAULT '0',
`is_show` tinyint(1) unsigned NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of think_group
-- ----------------------------
-- ----------------------------
-- Table structure for `think_menu`
-- ----------------------------
DROP TABLE IF EXISTS `think_menu`;
CREATE TABLE `think_menu` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`parent_id` int(11) NOT NULL COMMENT '上级ID',
`child_ids` varchar(128) NOT NULL COMMENT '下级ID用'',''分开',
`title` varchar(12) NOT NULL COMMENT '菜单名',
`position` varchar(8) NOT NULL COMMENT '菜单所在的位置top1,top2,menu,main',
`target` varchar(10) NOT NULL COMMENT '连接打开的位置header-frame,menu-framemain-frame,_blank',
`url` varchar(512) NOT NULL COMMENT '连接地址,有下级的用#或者JS来操作,连接从根开始,不带域名',
`app` varchar(24) NOT NULL COMMENT '所属分组应用,主要会控制左边的菜单变化,空为所有APP都可以',
`module` varchar(24) NOT NULL COMMENT '所属模块,空为做公共的',
`action` varchar(24) NOT NULL COMMENT '所属操作,空为公共的',
`node_id` int(11) NOT NULL COMMENT '标记对应的节点,创建应用、模块或操作时生成上下级的菜单',
`access_node` varchar(128) NOT NULL COMMENT '此菜单需要访问的节点,角色有此菜单时必需要给这些节点赋权,每个节点用,分开',
`group_id` tinyint(4) NOT NULL COMMENT '对菜单进行分组,这样不同的应用就可以按组来读取菜单了',
`user_id` int(11) NOT NULL DEFAULT '0' COMMENT '这个菜单导航的用户,当为0时是公共的',
`role_id` varchar(64) NOT NULL DEFAULT '[1][2]' COMMENT '角色的菜单,当角色菜单生效时,user_id不生效',
`listorder` smallint(6) NOT NULL DEFAULT '0' COMMENT '排序,从小到大',
`status` tinyint(4) NOT NULL DEFAULT '1' COMMENT '1:显示,0:不显示,-1:禁用',
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 COMMENT='系统导航菜单,app,module,action用于生成菜单的位置,node_id为找出上级节点菜单作准备';
-- ----------------------------
-- Records of think_menu
-- ----------------------------
-- ----------------------------
-- Table structure for `think_node`
-- ----------------------------
DROP TABLE IF EXISTS `think_node`;
CREATE TABLE `think_node` (
`id` smallint(6) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(32) NOT NULL,
`title` varchar(50) DEFAULT NULL,
`status` tinyint(1) DEFAULT '0',
`remark` varchar(255) DEFAULT NULL,
`sort` smallint(6) unsigned DEFAULT NULL,
`pid` smallint(6) unsigned NOT NULL,
`level` tinyint(1) unsigned NOT NULL,
`group_id` tinyint(3) unsigned DEFAULT '0',
`role_id` varchar(200) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `level` (`level`),
KEY `pid` (`pid`),
KEY `status` (`status`),
KEY `name` (`name`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of think_node
-- ----------------------------
-- ----------------------------
-- Table structure for `think_role`
-- ----------------------------
DROP TABLE IF EXISTS `think_role`;
CREATE TABLE `think_role` (
`id` smallint(6) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(20) NOT NULL,
`pid` smallint(6) NOT NULL DEFAULT '1',
`status` tinyint(1) DEFAULT NULL,
`remark` varchar(255) DEFAULT NULL,
`create_time` datetime DEFAULT NULL,
`update_time` datetime DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `parentId` (`pid`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 COMMENT='1为EMT开发人员,2为系统管理员,其它的按groupID作为起数,100以内为系统预留的ID';
-- ----------------------------
-- Records of think_role
-- ----------------------------
-- ----------------------------
-- Table structure for `think_role_admin`
-- ----------------------------
DROP TABLE IF EXISTS `think_role_admin`;
CREATE TABLE `think_role_admin` (
`admin_id` int(11) NOT NULL,
`role_code` varchar(20) DEFAULT NULL,
PRIMARY KEY (`admin_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 ROW_FORMAT=FIXED;
-- ----------------------------
-- Records of think_role_admin
-- ----------------------------
-- ----------------------------
-- Table structure for `think_role_user`
-- ----------------------------
DROP TABLE IF EXISTS `think_role_user`;
CREATE TABLE `think_role_user` (
`role_id` mediumint(9) unsigned DEFAULT NULL,
`user_id` char(32) DEFAULT NULL,
KEY `group_id` (`role_id`),
KEY `user_id` (`user_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of think_role_user
-- ----------------------------
-- ----------------------------
-- Table structure for `think_table`
-- ----------------------------
DROP TABLE IF EXISTS `think_table`;
CREATE TABLE `think_table` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(64) NOT NULL COMMENT '表名',
`title` varchar(32) NOT NULL COMMENT '表中文名',
`remark` varchar(128) NOT NULL COMMENT '备注',
`status` tinyint(4) NOT NULL DEFAULT '1' COMMENT '状态,-1表示在回收站,1表示正常,可以增加其它状态',
`add_user_id` int(11) NOT NULL COMMENT '添加人ID',
`add_time` int(11) NOT NULL COMMENT '添加时间',
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 COMMENT='系统表管理';
-- ----------------------------
-- Records of think_table
-- ----------------------------
-- ----------------------------
-- Table structure for `wp_public`
-- ----------------------------
DROP TABLE IF EXISTS `wp_public`;
CREATE TABLE `wp_public` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT COMMENT '主键',
`uid` int(10) DEFAULT NULL COMMENT '用户ID',
`public_name` varchar(50) DEFAULT NULL COMMENT '公众号名称',
`public_id` varchar(100) DEFAULT NULL COMMENT '公众号原始id',
`wechat` varchar(100) DEFAULT NULL COMMENT '微信号',
`interface_url` varchar(255) DEFAULT NULL COMMENT '接口地址',
`headface_url` varchar(255) DEFAULT NULL COMMENT '公众号头像',
`area` varchar(50) DEFAULT NULL COMMENT '地区',
`addon_config` text COMMENT '插件配置',
`addon_status` text COMMENT '插件状态',
`token` varchar(100) DEFAULT NULL COMMENT 'Token',
`is_use` tinyint(2) DEFAULT '0' COMMENT '是否为当前公众号',
`type` char(10) DEFAULT '0' COMMENT '公众号类型',
`appid` varchar(255) DEFAULT NULL COMMENT 'AppID',
`secret` varchar(255) DEFAULT NULL COMMENT 'AppSecret',
`group_id` int(10) unsigned DEFAULT '0' COMMENT '等级',
`encodingaeskey` varchar(255) DEFAULT NULL COMMENT 'EncodingAESKey',
`tips_url` varchar(255) DEFAULT NULL COMMENT '提示关注公众号的文章地址',
`domain` varchar(30) DEFAULT NULL COMMENT '自定义域名',
`is_bind` tinyint(2) DEFAULT '0' COMMENT '是否为微信开放平台绑定账号',
`pay_appid` varchar(100) DEFAULT NULL,
`pay_secret` varchar(100) DEFAULT NULL,
`mch_id` varchar(100) DEFAULT NULL,
`sub_mch_id` varchar(100) DEFAULT NULL,
`mch_secret` varchar(200) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `token` (`token`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wp_public
-- ----------------------------
-- ----------------------------
-- Table structure for `wx_notity_pay`
-- ----------------------------
DROP TABLE IF EXISTS `wx_notity_pay`;
CREATE TABLE `wx_notity_pay` (
`notify_pay_id` int(11) NOT NULL AUTO_INCREMENT,
`return_code` varchar(32) DEFAULT NULL,
`return_msg` varchar(32) DEFAULT NULL,
`appid` varchar(32) DEFAULT NULL,
`mch_id` varchar(32) DEFAULT NULL,
`device_info` varchar(32) DEFAULT NULL,
`nonce_str` varchar(32) DEFAULT NULL,
`sign` varchar(32) DEFAULT NULL,
`result_code` varchar(32) DEFAULT NULL,
`err_code` varchar(32) DEFAULT NULL,
`err_code_des` varchar(255) DEFAULT NULL,
`openid` varchar(128) DEFAULT NULL,
`is_subscribe` varchar(2) DEFAULT NULL,
`trade_type` varchar(32) DEFAULT NULL,
`bank_type` varchar(32) DEFAULT NULL,
`total_fee` int(11) DEFAULT NULL,
`fee_type` varchar(32) DEFAULT NULL,
`cash_fee` int(11) DEFAULT NULL,
`cash_fee_type` varchar(32) DEFAULT NULL,
`coupon_fee` int(11) DEFAULT NULL,
`coupon_count` int(5) DEFAULT NULL,
`coupon_id_1` varchar(32) DEFAULT NULL,
`coupon_fee_1` int(11) DEFAULT NULL,
`transaction_id` varchar(32) DEFAULT NULL,
`out_trade_no` varchar(32) DEFAULT NULL,
`attach` varchar(255) DEFAULT NULL,
`time_end` float(32,0) DEFAULT NULL,
PRIMARY KEY (`notify_pay_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wx_notity_pay
-- ----------------------------
-- ----------------------------
-- Table structure for `wx_unifiedorder`
-- ----------------------------
DROP TABLE IF EXISTS `wx_unifiedorder`;
CREATE TABLE `wx_unifiedorder` (
`wx_order_id` int(11) NOT NULL AUTO_INCREMENT,
`appid` varchar(64) DEFAULT NULL,
`mch_id` varchar(64) DEFAULT NULL,
`device_info` varchar(64) DEFAULT NULL,
`nonce_str` varchar(64) DEFAULT NULL,
`sign` varchar(64) DEFAULT NULL,
`body` varchar(255) DEFAULT NULL,
`detail` varchar(500) DEFAULT NULL,
`attach` varchar(255) DEFAULT NULL,
`out_trade_no` varchar(64) DEFAULT NULL,
`fee_type` varchar(64) DEFAULT NULL,
`total_fee` int(11) DEFAULT NULL,
`spbill_create_ip` varchar(64) DEFAULT NULL,
`time_start` float(32,0) DEFAULT NULL,
`time_expire` float(32,0) DEFAULT NULL,
`goods_tag` varchar(32) DEFAULT NULL,
`notify_url` varchar(255) DEFAULT NULL,
`trade_type` varchar(64) DEFAULT NULL,
`product_id` varchar(64) DEFAULT NULL,
`limit_pay` varchar(64) DEFAULT NULL,
`openid` varchar(100) DEFAULT NULL,
`e_pay_status` smallint(5) DEFAULT NULL,
PRIMARY KEY (`wx_order_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 ROW_FORMAT=COMPACT;
-- ----------------------------
-- Records of wx_unifiedorder
-- ----------------------------
-- ----------------------------
-- Table structure for `wx_unifiedorder_result`
-- ----------------------------
DROP TABLE IF EXISTS `wx_unifiedorder_result`;
CREATE TABLE `wx_unifiedorder_result` (
`wx_order_result_id` int(11) NOT NULL AUTO_INCREMENT,
`return_code` varchar(64) DEFAULT NULL,
`return_msg` varchar(255) DEFAULT NULL,
`device_info` varchar(64) DEFAULT NULL,
`appid` varchar(64) DEFAULT NULL,
`mch_id` varchar(64) DEFAULT NULL,
`nonce_str` varchar(64) DEFAULT NULL,
`sign` varchar(64) DEFAULT NULL,
`result_code` varchar(64) DEFAULT NULL,
`prepay_id` varchar(64) DEFAULT NULL,
`trade_type` varchar(64) DEFAULT NULL,
`err_code` varchar(64) DEFAULT NULL,
`err_code_des` varchar(255) DEFAULT NULL,
PRIMARY KEY (`wx_order_result_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 ROW_FORMAT=COMPACT;
-- ----------------------------
-- Records of wx_unifiedorder_result
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_building`
-- ----------------------------
DROP TABLE IF EXISTS `wy_building`;
CREATE TABLE `wy_building` (
`building_id` int(11) NOT NULL AUTO_INCREMENT,
`building_code` varchar(20) DEFAULT NULL,
`building_name` varchar(50) DEFAULT NULL,
`building_unit_quantity` int(11) DEFAULT NULL,
`building_floor_quantity` int(11) DEFAULT NULL,
`building_house_quantity` int(11) DEFAULT NULL,
`building_community_id` int(11) DEFAULT NULL,
`building_house_type_id` int(11) DEFAULT NULL,
`building_remark` text,
PRIMARY KEY (`building_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_building
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_certificate`
-- ----------------------------
DROP TABLE IF EXISTS `wy_certificate`;
CREATE TABLE `wy_certificate` (
`certificate_id` int(11) NOT NULL AUTO_INCREMENT,
`homeowner_id` int(11) DEFAULT NULL,
`homeowner_name` varchar(255) DEFAULT NULL,
`deal_date` date DEFAULT NULL,
`end_date` date DEFAULT NULL,
`community_id` int(11) DEFAULT NULL,
`building_id` int(11) DEFAULT NULL,
`house_id` int(11) DEFAULT NULL,
`remark` text,
PRIMARY KEY (`certificate_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_certificate
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_community`
-- ----------------------------
DROP TABLE IF EXISTS `wy_community`;
CREATE TABLE `wy_community` (
`community_id` int(11) NOT NULL AUTO_INCREMENT,
`community_code` varchar(20) DEFAULT NULL,
`community_name` varchar(50) DEFAULT NULL,
`community_building_quantity` int(4) DEFAULT NULL,
`community_house_quantity` int(4) DEFAULT NULL,
`community_address` varchar(200) DEFAULT NULL,
`community_introduction` text,
`community_remark` text,
PRIMARY KEY (`community_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_community
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_company`
-- ----------------------------
DROP TABLE IF EXISTS `wy_company`;
CREATE TABLE `wy_company` (
`company_id` int(11) NOT NULL AUTO_INCREMENT,
`company_code` varchar(20) DEFAULT NULL,
`company_name` varchar(100) DEFAULT NULL,
`nature_id` int(11) DEFAULT NULL,
`group_id` int(11) DEFAULT NULL,
`linkman` varchar(20) DEFAULT NULL,
`mobile` varchar(20) DEFAULT NULL,
`office_tel` varchar(20) DEFAULT NULL,
`home_tel` varchar(20) DEFAULT NULL,
`fax` varchar(20) DEFAULT NULL,
`mail` varchar(20) DEFAULT NULL,
`msn` varchar(20) DEFAULT NULL,
`qq` varchar(20) DEFAULT NULL,
`weixin` varchar(20) DEFAULT NULL,
`industry_id` int(11) DEFAULT NULL,
`main_business` varchar(200) DEFAULT NULL COMMENT '主营业务',
`homepage` varchar(200) DEFAULT NULL,
`address` varchar(200) DEFAULT NULL,
`remark` text,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
PRIMARY KEY (`company_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_company
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_customer`
-- ----------------------------
DROP TABLE IF EXISTS `wy_customer`;
CREATE TABLE `wy_customer` (
`customer_id` int(11) NOT NULL AUTO_INCREMENT,
`customer_code` varchar(50) DEFAULT NULL,
`customer_name` varchar(200) DEFAULT NULL,
`member_level_id` int(11) DEFAULT NULL,
`customer_level_id` int(11) DEFAULT NULL,
`customer_type_id` int(11) DEFAULT NULL,
`customer_source_id` int(11) DEFAULT NULL,
`industry_id` int(11) DEFAULT NULL,
`community_id` int(11) DEFAULT NULL,
`building_id` int(11) DEFAULT NULL,
`house_id` int(11) DEFAULT NULL,
`tel` varchar(20) DEFAULT NULL,
`fax` varchar(20) DEFAULT NULL,
`email` varchar(50) DEFAULT NULL,
`homepage` varchar(100) DEFAULT NULL,
`postcode` varchar(10) DEFAULT NULL,
`address` varchar(200) DEFAULT NULL,
`detail` text,
`remark` text,
`create_date` datetime DEFAULT NULL,
`update_date` datetime DEFAULT NULL,
PRIMARY KEY (`customer_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_customer
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_customer_level`
-- ----------------------------
DROP TABLE IF EXISTS `wy_customer_level`;
CREATE TABLE `wy_customer_level` (
`customer_level_id` int(11) NOT NULL AUTO_INCREMENT,
`customer_level_code` varchar(20) DEFAULT NULL,
`customer_level_name` varchar(50) DEFAULT NULL,
`remark` text,
PRIMARY KEY (`customer_level_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_customer_level
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_customer_source`
-- ----------------------------
DROP TABLE IF EXISTS `wy_customer_source`;
CREATE TABLE `wy_customer_source` (
`customer_source_id` int(11) NOT NULL AUTO_INCREMENT,
`customer_source_code` varchar(20) DEFAULT NULL,
`customer_source_name` varchar(50) DEFAULT NULL,
`remark` text,
PRIMARY KEY (`customer_source_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_customer_source
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_customer_type`
-- ----------------------------
DROP TABLE IF EXISTS `wy_customer_type`;
CREATE TABLE `wy_customer_type` (
`customer_type_id` int(11) NOT NULL AUTO_INCREMENT,
`customer_type_code` varchar(20) DEFAULT NULL,
`customer_type_name` varchar(50) DEFAULT NULL,
`remark` text,
PRIMARY KEY (`customer_type_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_customer_type
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_expense`
-- ----------------------------
DROP TABLE IF EXISTS `wy_expense`;
CREATE TABLE `wy_expense` (
`expense_id` int(11) NOT NULL AUTO_INCREMENT,
`charge_method_id` int(11) DEFAULT NULL,
`homeowner_id` int(11) DEFAULT NULL,
`homeowner_name` varchar(50) DEFAULT NULL,
`community_id` int(11) DEFAULT NULL,
`house_id` int(11) DEFAULT NULL,
`building_id` int(11) DEFAULT NULL,
`start_date` date DEFAULT NULL,
`end_date` date DEFAULT NULL,
`previous_degree` double(10,4) DEFAULT NULL,
`now_degree` double(10,4) DEFAULT NULL,
`remark` text,
PRIMARY KEY (`expense_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_expense
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_group`
-- ----------------------------
DROP TABLE IF EXISTS `wy_group`;
CREATE TABLE `wy_group` (
`group_id` int(11) NOT NULL AUTO_INCREMENT,
`group_code` varchar(20) DEFAULT NULL,
`group_name` varchar(50) DEFAULT NULL,
`remark` text,
PRIMARY KEY (`group_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_group
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_home_owner`
-- ----------------------------
DROP TABLE IF EXISTS `wy_home_owner`;
CREATE TABLE `wy_home_owner` (
`homeowner_id` int(11) NOT NULL AUTO_INCREMENT,
`homeowner_name` varchar(50) DEFAULT NULL,
`homeowner_sex` smallint(1) DEFAULT NULL,
`homeowner_card_type` smallint(1) DEFAULT NULL,
`homeowner_home_date` date DEFAULT NULL,
`house_status` smallint(1) DEFAULT NULL,
`house_community_id` int(11) DEFAULT NULL,
`house_building_id` int(11) DEFAULT NULL,
`house_id` int(11) DEFAULT NULL,
`homeowner_idcard_no` varchar(50) DEFAULT NULL,
`homeowner_company` varchar(50) DEFAULT NULL,
`homeowner_tel` varchar(50) DEFAULT NULL,
`homeowner_mobile` varchar(50) DEFAULT NULL,
`homeowner_email` varchar(50) DEFAULT NULL,
`homeowner_qq` varchar(50) DEFAULT NULL,
`homeowner_weixin` varchar(50) DEFAULT NULL,
`homeowner_country` varchar(50) DEFAULT NULL,
`homeowner_province` varchar(50) DEFAULT NULL,
`homeowner_race` varchar(50) DEFAULT NULL,
`homeowner_contract_no` varchar(50) DEFAULT NULL,
`homeowner_address` varchar(50) DEFAULT NULL,
`homeowner_zip_code` varchar(50) DEFAULT NULL,
`homeowner_police_station` varchar(50) DEFAULT NULL,
`homeowner_bank_no` varchar(50) DEFAULT NULL,
`homeowner_pressing_link` varchar(50) DEFAULT NULL,
`homeowner_pressing_tel` varchar(50) DEFAULT NULL,
`homeowner_pressing_address` varchar(50) DEFAULT NULL,
`homeowner_job_position` varchar(50) DEFAULT NULL,
`homeowner_remark` text,
PRIMARY KEY (`homeowner_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_home_owner
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_home_owner_change`
-- ----------------------------
DROP TABLE IF EXISTS `wy_home_owner_change`;
CREATE TABLE `wy_home_owner_change` (
`change_id` int(11) NOT NULL AUTO_INCREMENT,
`previous_homeowner_id` int(11) DEFAULT NULL,
`previous_homeowner_name` varchar(50) DEFAULT NULL,
`now_homeowner_id` int(11) DEFAULT NULL,
`now_homeowner_name` varchar(50) DEFAULT NULL,
`community_id` int(11) DEFAULT NULL,
`building_id` int(11) DEFAULT NULL,
`house_id` int(11) DEFAULT NULL,
`change_date` date DEFAULT NULL,
`remark` text,
PRIMARY KEY (`change_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_home_owner_change
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_industry`
-- ----------------------------
DROP TABLE IF EXISTS `wy_industry`;
CREATE TABLE `wy_industry` (
`industry_id` int(11) NOT NULL AUTO_INCREMENT,
`industry_code` varchar(20) DEFAULT NULL,
`industry_name` varchar(50) DEFAULT NULL,
`remark` text,
PRIMARY KEY (`industry_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_industry
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_living`
-- ----------------------------
DROP TABLE IF EXISTS `wy_living`;
CREATE TABLE `wy_living` (
`living_id` int(11) NOT NULL AUTO_INCREMENT,
`living_date` date DEFAULT NULL,
`end_date` date DEFAULT NULL,
`community_id` int(11) DEFAULT NULL,
`building_id` int(11) DEFAULT NULL,
`house_id` int(11) DEFAULT NULL,
`homeowner_id` int(11) DEFAULT NULL,
`homeowner_name` varchar(50) DEFAULT NULL,
`remark` text,
PRIMARY KEY (`living_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_living
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_member_level`
-- ----------------------------
DROP TABLE IF EXISTS `wy_member_level`;
CREATE TABLE `wy_member_level` (
`member_level_id` int(11) NOT NULL AUTO_INCREMENT,
`member_level_code` varchar(20) DEFAULT NULL,
`member_level_name` varchar(50) DEFAULT NULL,
`remark` text,
PRIMARY KEY (`member_level_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_member_level
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_nature`
-- ----------------------------
DROP TABLE IF EXISTS `wy_nature`;
CREATE TABLE `wy_nature` (
`nature_id` int(11) NOT NULL AUTO_INCREMENT,
`nature_code` varchar(20) DEFAULT NULL,
`nature_name` varchar(50) DEFAULT NULL,
`remark` text,
PRIMARY KEY (`nature_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_nature
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_project_accept`
-- ----------------------------
DROP TABLE IF EXISTS `wy_project_accept`;
CREATE TABLE `wy_project_accept` (
`projectaccept_id` int(11) NOT NULL AUTO_INCREMENT,
`projectaccept_name` varchar(20) DEFAULT NULL,
`homeowner_id` int(11) DEFAULT NULL,
`homeowner_name` varchar(50) DEFAULT NULL,
`deal_date` date DEFAULT NULL,
`end_date` date DEFAULT NULL,
`community_id` int(11) DEFAULT NULL,
`building_id` int(11) DEFAULT NULL,
`house_id` int(11) DEFAULT NULL,
`remark` text,
PRIMARY KEY (`projectaccept_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 ROW_FORMAT=DYNAMIC;
-- ----------------------------
-- Records of wy_project_accept
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_repair`
-- ----------------------------
DROP TABLE IF EXISTS `wy_repair`;
CREATE TABLE `wy_repair` (
`repair_id` int(11) NOT NULL AUTO_INCREMENT,
`repair_name` varchar(255) DEFAULT NULL,
`deal_date` date DEFAULT NULL,
`end_date` date DEFAULT NULL,
`homeowner_id` int(11) DEFAULT NULL,
`homeowner_name` varchar(255) DEFAULT NULL,
`community_id` int(11) DEFAULT NULL,
`building_id` int(11) DEFAULT NULL,
`house_id` int(11) DEFAULT NULL,
`remark` text,
`evaluator` varchar(50) DEFAULT NULL,
`assess_remark` text,
`assess_date` datetime DEFAULT NULL,
`maintenance` varchar(50) DEFAULT NULL,
`result_remark` text,
`result_date` datetime DEFAULT NULL,
`berichten` varchar(50) DEFAULT NULL,
`berichten_remark` text,
`berichten_date` datetime DEFAULT NULL,
PRIMARY KEY (`repair_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_repair
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_setting_charge_method`
-- ----------------------------
DROP TABLE IF EXISTS `wy_setting_charge_method`;
CREATE TABLE `wy_setting_charge_method` (
`setting_charge_method_id` int(11) NOT NULL AUTO_INCREMENT,
`setting_charge_method_code` varchar(20) DEFAULT NULL,
`setting_charge_method_name` varchar(50) DEFAULT NULL,
`setting_charge_method_remark` text,
PRIMARY KEY (`setting_charge_method_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_setting_charge_method
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_setting_house`
-- ----------------------------
DROP TABLE IF EXISTS `wy_setting_house`;
CREATE TABLE `wy_setting_house` (
`house_id` int(11) NOT NULL AUTO_INCREMENT,
`house_name` varchar(20) DEFAULT NULL,
`house_unit_no` varchar(20) DEFAULT NULL,
`house_floor` int(4) DEFAULT NULL,
`house_status` int(4) DEFAULT NULL,
`house_community_id` int(11) DEFAULT NULL,
`house_building_id` int(11) DEFAULT NULL,
`house_type_id` int(11) DEFAULT NULL,
`house_direction_id` int(11) DEFAULT NULL,
`house_remark` text,
PRIMARY KEY (`house_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_setting_house
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_setting_house_direction`
-- ----------------------------
DROP TABLE IF EXISTS `wy_setting_house_direction`;
CREATE TABLE `wy_setting_house_direction` (
`setting_house_direction_id` int(11) NOT NULL AUTO_INCREMENT,
`setting_house_direction_code` varchar(20) DEFAULT NULL,
`setting_house_direction_name` varchar(50) DEFAULT NULL,
`setting_house_direction_remark` text,
PRIMARY KEY (`setting_house_direction_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_setting_house_direction
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_setting_house_structure`
-- ----------------------------
DROP TABLE IF EXISTS `wy_setting_house_structure`;
CREATE TABLE `wy_setting_house_structure` (
`setting_house_structure_id` int(11) NOT NULL AUTO_INCREMENT,
`setting_house_structure_code` varchar(20) DEFAULT NULL,
`setting_house_structure_name` varchar(50) DEFAULT NULL,
`setting_house_structure_remark` text,
PRIMARY KEY (`setting_house_structure_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_setting_house_structure
-- ----------------------------
-- ----------------------------
-- Table structure for `wy_setting_house_type`
-- ----------------------------
DROP TABLE IF EXISTS `wy_setting_house_type`;
CREATE TABLE `wy_setting_house_type` (
`setting_house_type_id` int(11) NOT NULL AUTO_INCREMENT,
`setting_house_type_code` varchar(20) DEFAULT NULL,
`setting_house_type_name` varchar(50) DEFAULT NULL,
`setting_house_type_remark` text,
PRIMARY KEY (`setting_house_type_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of wy_setting_house_type
-- ----------------------------
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/mapper/ArticleMapper.java
package com.ehais.figoarticle.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
import com.ehais.figoarticle.model.Article;
import com.ehais.figoarticle.model.ArticleExample;
public interface ArticleMapper {
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
@Select("select count(*) from article where #{field} = #{value} ")
int unique(@Param("field") String field, @Param("value") String value);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
@Select("select count(*) from article where #{field} = #{value} and store_id = #{store_id}")
int uniqueStore(@Param("field") String field, @Param("value") String value, @Param("store_id") Integer store_id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
long countByExample(ArticleExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
int deleteByExample(ArticleExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
int deleteByPrimaryKey(Long id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
int insert(Article record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
int insertSelective(Article record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
List<Article> selectByExampleWithBLOBs(ArticleExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
List<Article> selectByExample(ArticleExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
Article selectByPrimaryKey(Long id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
int updateByExampleSelective(@Param("record") Article record, @Param("example") ArticleExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
int updateByExampleWithBLOBs(@Param("record") Article record, @Param("example") ArticleExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
int updateByExample(@Param("record") Article record, @Param("example") ArticleExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
int updateByPrimaryKeySelective(Article record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
int updateByPrimaryKeyWithBLOBs(Article record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbggenerated Sun Mar 19 21:21:17 CST 2017
*/
int updateByPrimaryKey(Article record);
}<file_sep>/eh-weixin/src/main/java/org/ehais/weixin/model/OrderPayModel.java
package org.ehais.weixin.model;
import java.io.Serializable;
public class OrderPayModel implements Serializable{
private static final long serialVersionUID = 3888296789376591346L;
private Integer wxid;
private Integer store_id;//跟wxid二选一就可以了,微信支付使用wxid,其它系统支付使用store_id
private String realname;
private String mobile;
private String email;
private String guestbook;//留言
private String company;//公司
private String address;//地址
private String orderPaySource;//支付类型:'unionpay','alipay','paybill','weixin'
private Float amount; //金额,以分为单位
private Integer anonymous;//0:非匿名,1:匿名,不显示名称
private String orderPayTableName;//用于哪个类型的支付
private Long orderPayTableId;//用于哪个类型支付的编号
private String orderBody;//支付描述
private String trade_type;//微信支付类型[JSAPI]
private String orderSn;//订单编号
private String notifyUrl;//回调通知地址
public Integer getWxid() {
return wxid;
}
public void setWxid(Integer wxid) {
this.wxid = wxid;
}
public String getRealname() {
return realname;
}
public void setRealname(String realname) {
this.realname = realname;
}
public String getMobile() {
return mobile;
}
public void setMobile(String mobile) {
this.mobile = mobile;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getGuestbook() {
return guestbook;
}
public void setGuestbook(String guestbook) {
this.guestbook = guestbook;
}
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
public Float getAmount() {
return amount;
}
public void setAmount(Float amount) {
this.amount = amount;
}
public Integer getAnonymous() {
return anonymous;
}
public void setAnonymous(Integer anonymous) {
this.anonymous = anonymous;
}
public String getOrderPayTableName() {
return orderPayTableName;
}
public void setOrderPayTableName(String orderPayTableName) {
this.orderPayTableName = orderPayTableName;
}
public Long getOrderPayTableId() {
return orderPayTableId;
}
public void setOrderPayTableId(Long orderPayTableId) {
this.orderPayTableId = orderPayTableId;
}
public String getOrderBody() {
return orderBody;
}
public void setOrderBody(String orderBody) {
this.orderBody = orderBody;
}
public String getTrade_type() {
return trade_type;
}
public void setTrade_type(String trade_type) {
this.trade_type = trade_type;
}
public Integer getStore_id() {
return store_id;
}
public void setStore_id(Integer store_id) {
this.store_id = store_id;
}
public String getOrderSn() {
return orderSn;
}
public void setOrderSn(String orderSn) {
this.orderSn = orderSn;
}
public String getNotifyUrl() {
return notifyUrl;
}
public void setNotifyUrl(String notifyUrl) {
this.notifyUrl = notifyUrl;
}
public String getOrderPaySource() {
return orderPaySource;
}
public void setOrderPaySource(String orderPaySource) {
this.orderPaySource = orderPaySource;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
<file_sep>/eh-common/src/main/java/org/ehais/model/WxUsers.java
package org.ehais.model;
import java.math.BigDecimal;
import java.util.Date;
public class WxUsers {
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.user_id
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private Integer user_id;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.email
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String email;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.user_name
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String user_name;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.user_no
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String user_no;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.password
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String password;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.nickname
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String nickname;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.realname
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String realname;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.question
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String question;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.answer
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String answer;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.sex
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private short sex;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.birthday
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private Date birthday;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.reg_time
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private Date reg_time;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.last_login
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private Date last_login;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.last_ip
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String last_ip;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.visit_count
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private Short visit_count;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.mobile
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String mobile;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.is_validated
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private Byte is_validated;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.credit_line
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private BigDecimal credit_line;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.passwd_question
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String passwd_question;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.passwd_answer
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String passwd_answer;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.store_id
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private Integer store_id;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.face_image
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String face_image;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.openid
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String openid;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.subscribe
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private Integer subscribe;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_users.self_description
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
private String self_description;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.user_id
*
* @return the value of wx_users.user_id
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public Integer getUser_id() {
return user_id;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.user_id
*
* @param user_id the value for wx_users.user_id
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setUser_id(Integer user_id) {
this.user_id = user_id;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.email
*
* @return the value of wx_users.email
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getEmail() {
return email;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.email
*
* @param email the value for wx_users.email
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setEmail(String email) {
this.email = email;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.user_name
*
* @return the value of wx_users.user_name
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getUser_name() {
return user_name;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.user_name
*
* @param user_name the value for wx_users.user_name
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setUser_name(String user_name) {
this.user_name = user_name;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.user_no
*
* @return the value of wx_users.user_no
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getUser_no() {
return user_no;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.user_no
*
* @param user_no the value for wx_users.user_no
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setUser_no(String user_no) {
this.user_no = user_no;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.password
*
* @return the value of wx_users.password
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getPassword() {
return password;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.password
*
* @param password the value for wx_users.password
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setPassword(String password) {
this.password = password;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.nickname
*
* @return the value of wx_users.nickname
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getNickname() {
return nickname;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.nickname
*
* @param nickname the value for wx_users.nickname
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setNickname(String nickname) {
this.nickname = nickname;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.realname
*
* @return the value of wx_users.realname
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getRealname() {
return realname;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.realname
*
* @param realname the value for wx_users.realname
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setRealname(String realname) {
this.realname = realname;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.question
*
* @return the value of wx_users.question
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getQuestion() {
return question;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.question
*
* @param question the value for wx_users.question
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setQuestion(String question) {
this.question = question;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.answer
*
* @return the value of wx_users.answer
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getAnswer() {
return answer;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.answer
*
* @param answer the value for wx_users.answer
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setAnswer(String answer) {
this.answer = answer;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.sex
*
* @return the value of wx_users.sex
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public short getSex() {
return sex;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.sex
*
* @param sex the value for wx_users.sex
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setSex(short sex) {
this.sex = sex;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.birthday
*
* @return the value of wx_users.birthday
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public Date getBirthday() {
return birthday;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.birthday
*
* @param birthday the value for wx_users.birthday
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.reg_time
*
* @return the value of wx_users.reg_time
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public Date getReg_time() {
return reg_time;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.reg_time
*
* @param reg_time the value for wx_users.reg_time
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setReg_time(Date reg_time) {
this.reg_time = reg_time;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.last_login
*
* @return the value of wx_users.last_login
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public Date getLast_login() {
return last_login;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.last_login
*
* @param last_login the value for wx_users.last_login
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setLast_login(Date last_login) {
this.last_login = last_login;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.last_ip
*
* @return the value of wx_users.last_ip
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getLast_ip() {
return last_ip;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.last_ip
*
* @param last_ip the value for wx_users.last_ip
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setLast_ip(String last_ip) {
this.last_ip = last_ip;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.visit_count
*
* @return the value of wx_users.visit_count
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public Short getVisit_count() {
return visit_count;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.visit_count
*
* @param visit_count the value for wx_users.visit_count
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setVisit_count(Short visit_count) {
this.visit_count = visit_count;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.mobile
*
* @return the value of wx_users.mobile
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getMobile() {
return mobile;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.mobile
*
* @param mobile the value for wx_users.mobile
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setMobile(String mobile) {
this.mobile = mobile;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.is_validated
*
* @return the value of wx_users.is_validated
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public Byte getIs_validated() {
return is_validated;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.is_validated
*
* @param is_validated the value for wx_users.is_validated
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setIs_validated(Byte is_validated) {
this.is_validated = is_validated;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.credit_line
*
* @return the value of wx_users.credit_line
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public BigDecimal getCredit_line() {
return credit_line;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.credit_line
*
* @param credit_line the value for wx_users.credit_line
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setCredit_line(BigDecimal credit_line) {
this.credit_line = credit_line;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.passwd_question
*
* @return the value of wx_users.passwd_question
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getPasswd_question() {
return passwd_question;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.passwd_question
*
* @param passwd_question the value for wx_users.passwd_question
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setPasswd_question(String passwd_question) {
this.passwd_question = passwd_question;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.passwd_answer
*
* @return the value of wx_users.passwd_answer
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getPasswd_answer() {
return passwd_answer;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.passwd_answer
*
* @param passwd_answer the value for wx_users.passwd_answer
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setPasswd_answer(String passwd_answer) {
this.passwd_answer = passwd_answer;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.store_id
*
* @return the value of wx_users.store_id
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public Integer getStore_id() {
return store_id;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.store_id
*
* @param store_id the value for wx_users.store_id
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setStore_id(Integer store_id) {
this.store_id = store_id;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.face_image
*
* @return the value of wx_users.face_image
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getFace_image() {
return face_image;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.face_image
*
* @param face_image the value for wx_users.face_image
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setFace_image(String face_image) {
this.face_image = face_image;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.openid
*
* @return the value of wx_users.openid
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getOpenid() {
return openid;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.openid
*
* @param openid the value for wx_users.openid
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setOpenid(String openid) {
this.openid = openid;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.subscribe
*
* @return the value of wx_users.subscribe
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public Integer getSubscribe() {
return subscribe;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.subscribe
*
* @param subscribe the value for wx_users.subscribe
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setSubscribe(Integer subscribe) {
this.subscribe = subscribe;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_users.self_description
*
* @return the value of wx_users.self_description
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public String getSelf_description() {
return self_description;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_users.self_description
*
* @param self_description the value for wx_users.self_description
*
* @mbg.generated Tue Dec 06 02:21:19 CST 2016
*/
public void setSelf_description(String self_description) {
this.self_description = self_description;
}
}<file_sep>/school-weixin/src/main/java/com/ehais/schoolweixin/mapper/wxRepairMapper.java
package com.ehais.schoolweixin.mapper;
import com.ehais.schoolweixin.model.wxRepair;
import com.ehais.schoolweixin.model.wxRepairExample;
import java.util.List;
import org.apache.ibatis.annotations.Param;
public interface wxRepairMapper {
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_repair
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
int countByExample(wxRepairExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_repair
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
int deleteByExample(wxRepairExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_repair
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
int deleteByPrimaryKey(Integer id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_repair
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
int insert(wxRepair record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_repair
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
int insertSelective(wxRepair record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_repair
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
List<wxRepair> selectByExample(wxRepairExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_repair
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
wxRepair selectByPrimaryKey(Integer id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_repair
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
int updateByExampleSelective(@Param("record") wxRepair record, @Param("example") wxRepairExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_repair
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
int updateByExample(@Param("record") wxRepair record, @Param("example") wxRepairExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_repair
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
int updateByPrimaryKeySelective(wxRepair record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_repair
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
int updateByPrimaryKey(wxRepair record);
}<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/service/SpringTaskService.java
package com.ehais.hrlucene.service;
public interface SpringTaskService {
public void myTask();
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/Service/Impl/GoodiesAttrImpl.java
package com.ehais.figoarticle.Service.Impl;
import com.ehais.figoarticle.mapper.HaiGoodsAttrMapper;
import com.ehais.figoarticle.mapper.HaiGoodsMapper;
import com.ehais.figoarticle.model.*;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.ArrayList;
import java.util.List;
/**
* Created by stephen on 5/27/17.
* Service for
*/
@Service("GoodiesAttr")
public class GoodiesAttrImpl implements com.ehais.figoarticle.Service.GoodiesAttr {
@Autowired
private HaiGoodsAttrMapper haiGoodsAttrMapper;
@Autowired
private HaiGoodsMapper haiGoodsMapper;
private List<CusHaiGoodsAttr> attrItemList = new ArrayList<>();
@Override
public void transfer(){
HaiGoodsAttrExample goodattrexample=new HaiGoodsAttrExample();
//HaiGoodsAttrExample.Criteria c=goodattrexample.createCriteria();
goodattrexample.setOrderByClause("goods_id desc,attr_type asc");
List<HaiGoodsAttr> haigoodsattrList=haiGoodsAttrMapper.selectByExampleWithBLOBs(goodattrexample);
CusHaiGoodsAttr cha = new CusHaiGoodsAttr();
cha.goodsId=haigoodsattrList.get(0).getGoodsId();
for(HaiGoodsAttr goodItem:haigoodsattrList){
if(cha.goodsId.equals(goodItem.getGoodsId())){
}
else
{
//this.attrItemList.add(cha);
this.update_haigood(cha);
cha=null;
cha=new CusHaiGoodsAttr();
cha.goodsId=goodItem.getGoodsId();
}
try {
switch (goodItem.getAttrType()) {
case "color": {
cha.colors.add(goodItem.getAttrValue());
}
break;
case "size": {
cha.sizes.add(goodItem.getAttrValue());
}
break;
default: {
System.out.println("unknow attr_type");
}
break;
}
}catch (Exception e){
e.printStackTrace();
}
}
}
private void update_haigood(CusHaiGoodsAttr item){
//prepare data
String attrgroup="";
attrgroup=attrgroup+"颜色:";
for(int i=0;i<item.colors.size();i++){
attrgroup+=item.colors.get(i);
if(i!=item.colors.size()-1){
attrgroup+=",";
}
}
attrgroup=attrgroup+"|尺寸:";
for(int j=0;j<item.sizes.size();j++){
attrgroup+=item.sizes.get(j);
if(j!=item.sizes.size()-1){
attrgroup+=",";
}
}
//To database
HaiGoodsWithBLOBs haiGoodsWithBLOBs = new HaiGoodsWithBLOBs();
HaiGoodsExample haiGoodsExample = new HaiGoodsExample();
haiGoodsExample.createCriteria().andGoodsIdEqualTo(item.goodsId);
haiGoodsWithBLOBs.setAttrGroup(attrgroup);
haiGoodsMapper.updateByExampleSelective(haiGoodsWithBLOBs,haiGoodsExample);
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/admin/SpiderController.java
package com.ehais.figoarticle.controller.admin;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.ehais.tools.ReturnObject;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.controller.FarfetchController;
import com.ehais.figoarticle.controller.FigoCommonController;
import com.ehais.figoarticle.controller.FlannelsController;
import com.ehais.figoarticle.controller.SsenseController;
import com.ehais.figoarticle.model.HaiWebsite;
import com.ehais.figoarticle.model.WebSiteCount;
@Controller
@RequestMapping("/spider")
public class SpiderController extends FigoCommonController {
@RequestMapping("/index")
public String cate(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
List<HaiWebsite> websiteList = haiWebsiteMapper.selectByExample(null);
modelMap.addAttribute("websiteList", websiteList);
return "/admin/spider";
}
@ResponseBody
@RequestMapping("website_count")
public String website_count(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
ReturnObject<Object> rm = new ReturnObject<Object>();
List<WebSiteCount> categoryWebSiteCount = haiCategoryMapper.groupWebSite();
List<WebSiteCount> goodsUrlWebSiteCount = haiGoodsUrlMapper.groupWebSite();
List<WebSiteCount> goodsWebSiteCount = haiGoodsMapper.groupWebSite();
Map<String,Object> map = new HashMap<String, Object>();
map.put("categoryWebSiteCount", categoryWebSiteCount);
map.put("goodsUrlWebSiteCount", goodsUrlWebSiteCount);
map.put("goodsWebSiteCount", goodsWebSiteCount);
rm.setMap(map);
rm.setCode(1);
return this.writeJson(rm);
}
@RequestMapping("/flannels")
public String flannels(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response
){
return "/admin/flannels";
}
@ResponseBody
@RequestMapping("/flannels-category")
public String flannelsCategory(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "content", required = true) String content
){
FlannelsController f = new FlannelsController();
f.category_content(request, content);
return "";
}
@RequestMapping("/farfetch")
public String farfetch(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response
){
return "/admin/farfetch";
}
@ResponseBody
@RequestMapping("/farfetch-category")
public String farfetchCategory(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "content", required = true) String content
){
FarfetchController f = new FarfetchController();
f.category_content(request, content);
return "";
}
@RequestMapping("/ssense")
public String ssense(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response
){
return "/admin/ssense";
}
@ResponseBody
@RequestMapping("/ssense-category")
public String ssenseCategory(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "content", required = true) String content
){
SsenseController f = new SsenseController();
f.category_content(request, content);
return "";
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/MytheresaController.java
package com.ehais.figoarticle.controller;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.ehais.util.Bean2Utils;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.model.HaiCategory;
import com.ehais.figoarticle.model.HaiCategoryExample;
import com.ehais.figoarticle.model.HaiGoodsAttr;
import com.ehais.figoarticle.model.HaiGoodsEntity;
import com.ehais.figoarticle.model.HaiGoodsGallery;
import com.ehais.figoarticle.model.HaiGoodsUrl;
import com.ehais.figoarticle.model.HaiGoodsUrlExample;
import com.ehais.figoarticle.model.HaiGoodsWithBLOBs;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
//完成
@Controller
@RequestMapping("/mytheresa")
public class MytheresaController extends FigoCommonController{
private static String url = "https://www.mytheresa.com";
private int websiteId = 14;
@ResponseBody
@RequestMapping("/brand")
public String brand(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
return "";
}
@ResponseBody
@RequestMapping("/category")
public String category(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
this.category(request, url + "/int_en/");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private void category(HttpServletRequest request,String categoryUrl){
String result = "";
try {
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), categoryUrl);
// result = PythonUtil.python("D:/workspace_jee/figoarticle/src/main/webapp/getAjaxWeb.py", categoryUrl);
// result = FSO.ReadFileName("E:/temp/IFCHIC.htm");
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(categoryUrl).timeout(10000).get();
List<HaiCategory> list = new ArrayList<HaiCategory>();
Element nav = doc.select(".nav-primary").first();
Elements navli = nav.select("li.level0");
for (Element element : navli) {
Element parent = element.select("a.level0.has-children").first();
System.out.println(parent.text());
HaiCategory pCate = new HaiCategory();
pCate.setCatName(parent.text());
pCate.setCategoryUrl(parent.attr("href"));
pCate.setIsShow(true);
pCate.setWebsiteId(websiteId);
Elements childrenLi = element.select("a.level1");
List<HaiCategory> children = new ArrayList<>();
for (Element element1 : childrenLi) {
String chil = element1.text();
System.out.println(chil);
String cHref = element1.attr("href");
HaiCategory cCate = new HaiCategory();
cCate.setCatName(element1.text());
cCate.setCategoryUrl(cHref);
cCate.setIsShow(true);
cCate.setWebsiteId(websiteId);
children.add(cCate);
}
pCate.setChildren(children);
list.add(pCate);
}
System.out.println("==========================================");
System.out.println("==========================================");
JSONArray arr = JSONArray.fromObject(list);
System.out.println(arr.toString());
// System.out.println(element.html());
System.out.println("==========================================");
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/category";
// String api = "http://localhost:8087/api/category";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
}
@ResponseBody
@RequestMapping("/url")
public String url(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
c.andCategoryUrlLike(url+"%");
List<HaiCategory> listCategory = haiCategoryMapper.selectByExample(example);
for (HaiCategory haiCategory : listCategory) {
// System.out.println(haiCategory.getCategoryUrl());
this.goodsUrl(request, haiCategory.getCategoryUrl(), haiCategory.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsUrl(HttpServletRequest request,String goodsurl,Integer catId){
System.out.println("请求地址:" + goodsurl);
String result = "";
try{
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
// result = PythonUtil.python("D:/workspace_jee/figoarticle/src/main/webapp/getAjaxWeb.py", categoryUrl);
// result = FSO.ReadFileName("E:/temp/IFCHIC.htm");
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(goodsurl).timeout(10000).get();
Element prolist = doc.select(".products-grid").first();
if (prolist == null || prolist.equals("")) return "";
Elements proli = prolist.select("li.item");
List<String> list = new ArrayList<String>();
for (Element element : proli) {
String href = element.select("a").first().attr("href");
System.out.println(href);
list.add(href);
}
JSONArray arr = JSONArray.fromObject(list);
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("catId", catId.toString());
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/url";
// String api = "http://localhost:8087/api/url";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
//获取下一页
Element next = doc.select("li.next").first();
if (next != null) {
String nHref = next.select("a").attr("href");
this.goodsUrl(request, nHref, catId);
}
}catch(Exception e){
e.printStackTrace();
}
return "";
}
@ResponseBody
@RequestMapping("/goodsAll")
public String goodsAll(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiGoodsUrlExample example = new HaiGoodsUrlExample();
HaiGoodsUrlExample.Criteria c = example.createCriteria();
c.andGoodsUrlLike(url+"%");
c.andFinishEqualTo(false);
List<HaiGoodsUrl> listGoodsUrl = haiGoodsUrlMapper.selectByExample(example);
for (HaiGoodsUrl haiGoodsUrl : listGoodsUrl) {
goodsModel(request,haiGoodsUrl.getGoodsUrl(),haiGoodsUrl.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
public String goodsModel(HttpServletRequest request , String goodsurl ,Integer catId){
System.out.println(goodsurl);
String result = "";
HaiGoodsEntity entity = new HaiGoodsEntity();
HaiGoodsWithBLOBs goods = new HaiGoodsWithBLOBs();
List<HaiGoodsGallery> goodsGalleryList = new ArrayList<HaiGoodsGallery>();
List<HaiGoodsAttr> goodsAttrList = new ArrayList<HaiGoodsAttr>();
try{
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(goodsurl).timeout(10000).get();
String name = doc.select(".product-name").first().text();
String sPrice = "";
Elements sale = doc.select(".special-price");
if (sale.size() == 0) {
sPrice = doc.select(".regular-price").first().text().substring(2);
} else {
sPrice = sale.first().select(".price").first().text().substring(2);
}
sPrice = sPrice.replace(",", "");
Integer price = Float.valueOf(sPrice).intValue() * 100;
String desc = doc.select(".product-description").first().text();
String currency = doc.select(".price").first().text().substring(0,1);
goods.setGoodsName(name);
goods.setShopPrice(price);
goods.setGoodsDesc(desc);
goods.setCurrency(currency);
goods.setGoodsUrl(goodsurl);
goods.setCatId(catId);
goods.setWebsiteId(websiteId);
//picture
Elements gallery_li = doc.select("[id^=image-]");
for (Element element : gallery_li) {
String gHref = element.attr("src");
gHref = "http:" + gHref;
HaiGoodsGallery gallery = new HaiGoodsGallery();
gallery.setThumbUrl(gHref);
gallery.setImgUrl(gHref);
gallery.setImgOriginal(gHref);
goodsGalleryList.add(gallery);
}
HaiGoodsGallery gallery = goodsGalleryList.get(0);
goods.setGoodsThumb(gallery.getThumbUrl());
goods.setGoodsImg(gallery.getImgUrl());
goods.setOriginalImg(gallery.getImgOriginal());
//size
Element sizes = doc.select("ul.sizes").first();
if (sizes != null) {
Elements size = sizes.select("li");
List<String> sizeList = new ArrayList<>();
for (Element element : size) {
HaiGoodsAttr goodsSize = new HaiGoodsAttr();
goodsSize.setAttrType("size");
goodsSize.setAttrPrice(price.toString());
Element sizea = element.select("a").first();
if (sizea.select("span").isEmpty()) {
goodsSize.setAttrValue(sizea.text());
} else {
goodsSize.setAttrValue(sizea.select("span").first().text());
}
sizeList.add(goodsSize.getAttrValue());
goodsAttrList.add(goodsSize);
}
}
Bean2Utils.printEntity(goods);
entity.setGoods(goods);
entity.setGoodsAttrList(goodsAttrList);
entity.setGoodsGalleryList(goodsGalleryList);
JSONObject jsonObject = JSONObject.fromObject(entity);
System.out.println(jsonObject.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", jsonObject.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/goodsAttr";
// String api = "http://localhost:8087/api/goods";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
//单商品的地址请求
@ResponseBody
@RequestMapping("/getGoodsUrl")
public String getGoodsUrl(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "catId", required = true) Integer catId,
@RequestParam(value = "goodsurl", required = true) String goodsurl
){
try{
return goodsModel(request,goodsurl,catId);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
public static void main(String[] args) throws Exception {
String goodsurl = "https://www.net-a-porter.com/cn/zh/d/Shop/Lingerie/All?cm_sp=topnav-_-clothing-_-lingerie";
DemoController ac = new DemoController();
// ac.goodsModel(url,1);
// ac.goodsUrl(null, goodsurl, 1);
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/mapper/ArticleCommentMapper.java
package com.ehais.figoarticle.mapper;
import com.ehais.figoarticle.model.ArticleComment;
import com.ehais.figoarticle.model.ArticleCommentExample;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.ResultMap;
import org.apache.ibatis.annotations.Select;
public interface ArticleCommentMapper {
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
@Select("select count(*) from article_comment where #{field} = #{value} ")
int unique(@Param("field") String field, @Param("value") String value);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
@Select("select count(*) from article_comment where #{field} = #{value} and store_id = #{store_id}")
int uniqueStore(@Param("field") String field, @Param("value") String value, @Param("store_id") Integer store_id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
long countByExample(ArticleCommentExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
int deleteByExample(ArticleCommentExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
int deleteByPrimaryKey(Integer orderId);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
int insert(ArticleComment record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
int insertSelective(ArticleComment record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
List<ArticleComment> selectByExample(ArticleCommentExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
ArticleComment selectByPrimaryKey(Integer orderId);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
int updateByExampleSelective(@Param("record") ArticleComment record, @Param("example") ArticleCommentExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
int updateByExample(@Param("record") ArticleComment record, @Param("example") ArticleCommentExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
int updateByPrimaryKeySelective(ArticleComment record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article_comment
*
* @mbg.generated Sat Jun 10 10:48:39 CST 2017
*/
int updateByPrimaryKey(ArticleComment record);
}<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/model/HaiHrCompany.java
package com.ehais.hrlucene.model;
public class HaiHrCompany {
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.company_id
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private Long company_id;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.company_name
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String company_name;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.company_website
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String company_website;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.company_industry
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String company_industry;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.company_scale
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String company_scale;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.company_nature
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String company_nature;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.welfare
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String welfare;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.company_address
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String company_address;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.company_email
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String company_email;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.company_fax
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String company_fax;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.contactperson
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String contactperson;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.tel
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String tel;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.phone
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String phone;
/**
* This field was generated by MyBatis Generator. This field corresponds to the database column hai_hr_company.company_detail
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
private String company_detail;
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.company_id
* @return the value of hai_hr_company.company_id
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public Long getCompany_id() {
return company_id;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.company_id
* @param company_id the value for hai_hr_company.company_id
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setCompany_id(Long company_id) {
this.company_id = company_id;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.company_name
* @return the value of hai_hr_company.company_name
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getCompany_name() {
return company_name;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.company_name
* @param company_name the value for hai_hr_company.company_name
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setCompany_name(String company_name) {
this.company_name = company_name;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.company_website
* @return the value of hai_hr_company.company_website
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getCompany_website() {
return company_website;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.company_website
* @param company_website the value for hai_hr_company.company_website
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setCompany_website(String company_website) {
this.company_website = company_website;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.company_industry
* @return the value of hai_hr_company.company_industry
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getCompany_industry() {
return company_industry;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.company_industry
* @param company_industry the value for hai_hr_company.company_industry
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setCompany_industry(String company_industry) {
this.company_industry = company_industry;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.company_scale
* @return the value of hai_hr_company.company_scale
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getCompany_scale() {
return company_scale;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.company_scale
* @param company_scale the value for hai_hr_company.company_scale
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setCompany_scale(String company_scale) {
this.company_scale = company_scale;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.company_nature
* @return the value of hai_hr_company.company_nature
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getCompany_nature() {
return company_nature;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.company_nature
* @param company_nature the value for hai_hr_company.company_nature
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setCompany_nature(String company_nature) {
this.company_nature = company_nature;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.welfare
* @return the value of hai_hr_company.welfare
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getWelfare() {
return welfare;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.welfare
* @param welfare the value for hai_hr_company.welfare
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setWelfare(String welfare) {
this.welfare = welfare;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.company_address
* @return the value of hai_hr_company.company_address
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getCompany_address() {
return company_address;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.company_address
* @param company_address the value for hai_hr_company.company_address
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setCompany_address(String company_address) {
this.company_address = company_address;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.company_email
* @return the value of hai_hr_company.company_email
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getCompany_email() {
return company_email;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.company_email
* @param company_email the value for hai_hr_company.company_email
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setCompany_email(String company_email) {
this.company_email = company_email;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.company_fax
* @return the value of hai_hr_company.company_fax
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getCompany_fax() {
return company_fax;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.company_fax
* @param company_fax the value for hai_hr_company.company_fax
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setCompany_fax(String company_fax) {
this.company_fax = company_fax;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.contactperson
* @return the value of hai_hr_company.contactperson
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getContactperson() {
return contactperson;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.contactperson
* @param contactperson the value for hai_hr_company.contactperson
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setContactperson(String contactperson) {
this.contactperson = contactperson;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.tel
* @return the value of hai_hr_company.tel
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getTel() {
return tel;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.tel
* @param tel the value for hai_hr_company.tel
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setTel(String tel) {
this.tel = tel;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.phone
* @return the value of hai_hr_company.phone
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getPhone() {
return phone;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.phone
* @param phone the value for hai_hr_company.phone
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setPhone(String phone) {
this.phone = phone;
}
/**
* This method was generated by MyBatis Generator. This method returns the value of the database column hai_hr_company.company_detail
* @return the value of hai_hr_company.company_detail
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public String getCompany_detail() {
return company_detail;
}
/**
* This method was generated by MyBatis Generator. This method sets the value of the database column hai_hr_company.company_detail
* @param company_detail the value for hai_hr_company.company_detail
* @mbg.generated Sun Nov 27 14:17:29 CST 2016
*/
public void setCompany_detail(String company_detail) {
this.company_detail = company_detail;
}
}<file_sep>/reptileV1/src/main/java/com/ehais/reptile/ReptileThread.java
package com.ehais.reptile;
import java.util.List;
import java.util.Map;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Text;
import org.ehais.tools.ReturnObject;
import com.ehais.bean.Goods;
import com.ehais.http.HttpGoods;
import com.ehais.reptile.impl.MytheresaImpl;
import com.ehais.reptile.impl.ShopBopImpl;
public class ReptileThread extends Thread {
private ShopBopImpl shopbop;
private MytheresaImpl mytheresa;
private String url;
private Integer type;
private ReturnObject<Object> rm;
private Text text_result;
private Goods goods;
private HttpGoods httpGoods;
private String result;
private Display display;
public ReptileThread(Display display,Text text_result) {
// TODO Auto-generated constructor stub
shopbop = new ShopBopImpl();
mytheresa = new MytheresaImpl();
url = "";
type = 0;
rm = new ReturnObject<Object>();
httpGoods = new HttpGoods();
this.text_result = text_result;
this.display = display;
}
public void setUrl(String url){
this.url = url;
}
public void setType(Integer type){
this.type = type;
}
public void run(){
try{
if(Thread.currentThread().getName().equals("shopbop")){
if(type == 0){
rm = shopbop.product_list(url);
}else{
rm = shopbop.product_info(url);
}
}else if(Thread.currentThread().getName().equals("mytheresa")){
}
if(rm!=null){
if(type == 0){
List<Object> list = rm.getRows();
for (Object object : list) {
Map<String,String> map = (Map<String, String>) object;
text_result.setText(text_result.getText()+ "获取商品:"+map.get("title")+"\r\n");
}
}else{
goods = (Goods) rm.getModel();
goods.setCategoryName("总分类");
text_result.setText(text_result.getText()+ "名称:"+goods.getGoodsName()+"\r\n");
text_result.setText(text_result.getText()+ "价格:"+goods.getPrice()+"\r\n");
text_result.setText(text_result.getText()+ "品牌:"+goods.getBrandName()+"\r\n");
text_result.setText(text_result.getText()+ "颜色:"+goods.getColorJson()+"\r\n");
text_result.setText(text_result.getText()+ "尺寸:"+goods.getSizeJson()+"\r\n");
result = httpGoods.postGoods(goods);
text_result.setText(text_result.getText()+ "提交结果:"+result+"\r\n");
}
}
display.asyncExec(this);
}catch(Exception e){
e.printStackTrace();
//需要补充上传错误信息
}
}
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/TessabitController.java
package com.ehais.figoarticle.controller;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.ehais.util.Bean2Utils;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.model.HaiCategory;
import com.ehais.figoarticle.model.HaiCategoryExample;
import com.ehais.figoarticle.model.HaiGoodsAttr;
import com.ehais.figoarticle.model.HaiGoodsEntity;
import com.ehais.figoarticle.model.HaiGoodsGallery;
import com.ehais.figoarticle.model.HaiGoodsUrl;
import com.ehais.figoarticle.model.HaiGoodsUrlExample;
import com.ehais.figoarticle.model.HaiGoodsWithBLOBs;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
@Controller
@RequestMapping("/tessabit")
public class TessabitController extends FigoCommonController{
//用于爬取分类
private static String url = "https://www.tessabit.com/cn/woman/";
//用于 tessabit/url goodsAll
private static String url2 = "https://www.tessabit.com/";
private static String url3 = "https://www.tessabit.com/cn/man/";
private int websiteId = 21;
@ResponseBody
@RequestMapping("/brand")
public String brand(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
return "";
}
@ResponseBody
@RequestMapping("/category")
public String category(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
this.category(request, url);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private void category(HttpServletRequest request,String categoryUrl){
String result = "";
try {
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), categoryUrl);
// result = GetPostTest.sendGet(categoryUrl, "country=CN");
Document doc = Jsoup.parse(result);
List<HaiCategory> list = new ArrayList<HaiCategory>();
// TODO
// System.out.println(doc);
//得到主菜单的 div
Element mainMenu = doc.select(".main-menu.text-center.hidden-xs.hidden-ms.hidden-sm.col-sm-6").first();
//得到菜单项
Elements menuLis = mainMenu.getElementsByTag("ul").first().select(">li");
String parentHref = "";
//遍历主菜单
for(Element menuLi : menuLis) {
Element menuLiA = menuLi.select(">a").first();
System.out.println(menuLiA.text());
if(menuLiA.text().equals("The Edit") || menuLiA.text().equals("Boutiques"))
continue;
parentHref = menuLiA.attr("href");
System.out.println(parentHref);
HaiCategory hc = new HaiCategory();
hc.setCatName(menuLiA.text());
hc.setCategoryUrl(parentHref);
hc.setWebsiteId(websiteId);
hc.setIsShow(true);
Element colDiv = menuLi.getElementsByClass("col-sm-4").first();
Element ul = colDiv.getElementsByTag("ul").first();
Elements catLis = ul.select(">li");
List<HaiCategory> catList = new ArrayList<HaiCategory>();
for(Element catLi : catLis) {
Element la = catLi.getElementsByTag("a").first();
System.out.println(la.attr("href"));
if(la.text().contains("Designers"))
continue;
HaiCategory hc1 = new HaiCategory();
hc1.setCatName(la.text());
hc1.setCategoryUrl(la.attr("href"));
hc1.setWebsiteId(websiteId);
hc1.setIsShow(true);
catList.add(hc1);
}
hc.setChildren(catList);
list.add(hc);
}
System.out.println("==========================================");
System.out.println("==========================================");
JSONArray arr = JSONArray.fromObject(list);
System.out.println(arr.toString());
// System.out.println(element.html());
System.out.println("==========================================");
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", arr.toString());
// String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/category";
String api = "http://localhost:8080/api/category";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
}
@ResponseBody
@RequestMapping("/url")
public String url(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
c.andCategoryUrlLike(url2+"%");
List<HaiCategory> listCategory = haiCategoryMapper.selectByExample(example);
for (HaiCategory haiCategory : listCategory) {
System.out.println(haiCategory.getCategoryUrl());
this.goodsUrl(request, haiCategory.getCategoryUrl(), haiCategory.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsUrl(HttpServletRequest request,String goodsurl,Integer catId){
System.out.println(goodsurl);
String result = "";
try{
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
Document doc = Jsoup.parse(result);
// result = GetPostTest.sendGet(goodsurl, null);
// Document doc = Jsoup.parse(result);
if(doc == null)
return "";
List<String> list = new ArrayList<String>();
// TODO
Element categoryProducts = doc.getElementsByClass("category-products").first();
if(categoryProducts == null)
return "";
//System.out.println(categoryProducts);
Element ul = categoryProducts.select(".products-grid.grid-mode.row-columns-4").first();
// System.out.println(ul);
Elements lisProduct = ul.getElementsByTag("li");
for(Element liProduct : lisProduct) {
Element lia = liProduct.getElementsByTag("a").first();
System.out.println(lia.attr("href"));
if(lia.attr("href") != null)
list.add(lia.attr("href"));
}
JSONArray arr = JSONArray.fromObject(list);
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("catId", catId.toString());
paramsMap.put("json", arr.toString());
// String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/url";
String api = "http://localhost:8080/api/url";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
/* Element pageIndex = doc.getElementsByClass("selectric").first();
Element optionIndex = pageIndex.getElementsByTag("option").first();
if(optionIndex == null)
return "";
int index = Integer.parseInt(optionIndex.text());
System.out.println("index = " + index);
if(index == 1) {
String pUrl = goodsurl + "?p=";
Element pager = doc.getElementsByClass("pager-container").first();
//System.out.println(pager);
Element spanNumber = pager.getElementsByClass("number").first();
int pagerNum = Integer.parseInt(spanNumber.text());
for(int i = 2; i <= pagerNum; i++) {
System.out.println("page = " + i);
this.goodsUrl(request,pUrl + i,catId);
}
}*/
Element pager = doc.getElementsByClass("pager").first();
// System.out.println(pager);
Element nextdisabled = pager.select(".next_jump.disabled").first();
if(nextdisabled == null) {
Element next = pager.getElementsByClass("next_jump").first();
if(next != null) {
System.out.println(next.attr("href"));
this.goodsUrl(request, next.attr("href"),catId);
}
} else {
System.out.println("最后一页了!");
return "";
}
//获取下一页
/**
Element page_chooser = page.getElementById("page-chooser");
Element next = page_chooser.getElementsByClass("next").first();
if(next != null){
String href_a = next.attr("href");
this.goodsUrl(request, href_a, catId);
}
**/
}catch(Exception e){
e.printStackTrace();
}
return result;
}
@ResponseBody
@RequestMapping("/goodsAll")
public String goodsAll(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiGoodsUrlExample example = new HaiGoodsUrlExample();
HaiGoodsUrlExample.Criteria c = example.createCriteria();
c.andGoodsUrlLike(url2+"%");
c.andFinishEqualTo(false);
List<HaiGoodsUrl> listGoodsUrl = haiGoodsUrlMapper.selectByExample(example);
for (HaiGoodsUrl haiGoodsUrl : listGoodsUrl) {
goodsModel(request,haiGoodsUrl.getGoodsUrl(),haiGoodsUrl.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
public String goodsModel(HttpServletRequest request , String goodsurl ,Integer catId){
System.out.println(goodsurl);
String result = "";
HaiGoodsEntity entity = new HaiGoodsEntity();
HaiGoodsWithBLOBs goods = new HaiGoodsWithBLOBs();
List<HaiGoodsGallery> goodsGalleryList = new ArrayList<HaiGoodsGallery>();
List<HaiGoodsAttr> goodsAttrList = new ArrayList<HaiGoodsAttr>();
goods.setGoodsUrl(goodsurl);
goods.setCatId(catId);
goods.setWebsiteId(websiteId);
try{
//
// result = PythonUtil.python("C:/Users/cjc/Desktop/eh-project/FigoShop/getAjaxWeb.py", goodsurl);
//result = GetPostTest.sendGet(goodsurl, null);
// Document doc = Jsoup.parse(result);
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
Document doc = Jsoup.parse(result);
// System.out.println(doc);
if(doc == null)
return "";
//细节描述
Element detail = doc.getElementById("details-section");
if(detail == null)
return "";
// System.out.println(detail.toString());
goods.setGoodsDesc(detail.toString());
//商品
Element goodName = doc.getElementsByClass("product-name").first().getElementsByTag("h1").first();
System.out.println(goodName.text());
goods.setGoodsName(goodName.text());
goods.setCatId(catId);
goods.setGoodsUrl(goodsurl);
//设置价格
Integer price;
Element priceInfo = doc.getElementsByClass("price-info").first();
Element productPrice = priceInfo.getElementsByClass("special-price").first();
if(productPrice != null) {
Element priceSpan = productPrice.getElementsByClass("price").first();
System.out.println(priceSpan.text());
String priceStr = priceSpan.text();
String currency = priceStr.substring(0,3);
goods.setCurrency(currency);
if(priceStr.indexOf(',') < 0) {
price = Integer.parseInt(priceStr.substring(3,priceStr.length() - 1));
}
else {
int index = priceStr.indexOf(',');
int thousand = Integer.parseInt(priceStr.substring(3,index));
int hundred = Integer.parseInt(priceStr.substring(index + 1, priceStr.length() - 1));
price = thousand * 1000 + hundred;
}
goods.setShopPrice(price);
} else {
Element priceSpanParent = priceInfo.getElementsByClass("regular-price").first();
Element priceSpan = priceSpanParent.getElementsByClass("price").first();
System.out.println(priceSpan.text());
String priceStr = priceSpan.text();
System.out.println(priceStr);
String currency = priceStr.substring(0,3);
goods.setCurrency(currency);
if(priceStr.indexOf(',') < 0) {
price = Integer.parseInt(priceStr.substring(3,priceStr.length() - 1));
}
else {
int index = priceStr.indexOf(',');
int thousand = Integer.parseInt(priceStr.substring(3,index));
int hundred = Integer.parseInt(priceStr.substring(index + 1, priceStr.length() - 1));
price = thousand * 1000 + hundred;
}
goods.setShopPrice(price);
}
//设置颜色
Element descriptionDiv = doc.getElementsByClass("description").first();
String description = descriptionDiv.text();
int index = description.indexOf(' ');
String color = description.substring(0, index);
HaiGoodsAttr goodsColor = new HaiGoodsAttr();
goodsColor.setAttrValue(color);
goodsColor.setAttrType("color");
goodsColor.setAttrPrice(price.toString());
goodsAttrList.add(goodsColor);
//设置 size
Element sizeSelect = doc.select(".size-selector.col-xs-12").first();
Elements sizeLabels = sizeSelect.getElementsByTag("a");
System.out.println(sizeLabels);
for(Element sizeLabel : sizeLabels) {
if(sizeLabel != null) {
HaiGoodsAttr goodsAttr1 = new HaiGoodsAttr();
goodsAttr1.setAttrValue(sizeLabel.text());
goodsAttr1.setAttrType("size");
goodsAttr1.setAttrPrice(price.toString());
goodsAttrList.add(goodsAttr1);
}
}
//设置图片链接
Element row = doc.getElementById("product-gallery");
Element div = row.select(".product-gallery-carousel.owl-carousel.owl-theme.owl-loaded").first();
Elements imgDivs = div.select(".item.easyzoom.easyzoom--overlay.container-image");
System.out.println(imgDivs);
for(Element imgDiv : imgDivs) {
Element imgA = imgDiv.getElementsByClass("product-image").first();
Element responseImg = imgDiv.getElementsByTag("img").first();
System.out.println(responseImg);
HaiGoodsGallery gallery = new HaiGoodsGallery();
gallery.setThumbUrl(imgA.attr("href"));
gallery.setImgUrl(responseImg.attr("src"));
gallery.setImgOriginal(responseImg.attr("src"));
goodsGalleryList.add(gallery);
}
if(goodsGalleryList.size() > 0) {
HaiGoodsGallery gallery = goodsGalleryList.get(0);
goods.setGoodsThumb(gallery.getThumbUrl());
goods.setGoodsImg(gallery.getImgUrl());
goods.setOriginalImg(gallery.getImgOriginal());
}
// Bean2Utils.printEntity(goods);
entity.setGoods(goods);
entity.setGoodsAttrList(goodsAttrList);
entity.setGoodsGalleryList(goodsGalleryList);
JSONObject jsonObject = JSONObject.fromObject(entity);
System.out.println(jsonObject.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", jsonObject.toString());
String api = "http://localhost:8080/api/goodsAttr";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
//单商品的地址请求
@ResponseBody
@RequestMapping("/getGoodsUrl")
public String getGoodsUrl(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "catId", required = true) Integer catId,
@RequestParam(value = "goodsurl", required = true) String goodsurl
){
try{
return goodsModel(request,goodsurl,catId);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
public static void main(String[] args) throws Exception {
String goodsurl = "https://www.net-a-porter.com/cn/zh/d/Shop/Lingerie/All?cm_sp=topnav-_-clothing-_-lingerie";
DemoController ac = new DemoController();
// ac.goodsModel(url,1);
// ac.goodsUrl(null, goodsurl, 1);
}
}
<file_sep>/FigoShop/src/main/webapp/getAjaxWeb.py
# -*- coding: utf-8 -*-
import sys
import urllib
import time
def getData (url):
if url != "":
from selenium import webdriver
driver = webdriver.PhantomJS(service_args=['--ignore-ssl-errors=true'])
driver.get(url)
time.sleep(3)
domStr=driver.execute_script("return document.documentElement.outerHTML")
sourcex=domStr.encode('utf-8')
driver.quit
sys.exit
return sourcex
else :
return ""
data = getData(sys.argv[1])
print data
<file_sep>/reptileV1/src/main/java/com/ehais/bean/Brand.java
package com.ehais.bean;
public class Brand {
private String brand_name;
private String brand_logo;
private String brand_desc;
private String site_url;
private String sort_order;
private String is_show;
private String store_id;
public Brand() {
// TODO Auto-generated constructor stub
}
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
}
public String getBrand_name() {
return brand_name;
}
public void setBrand_name(String brand_name) {
this.brand_name = brand_name;
}
public String getBrand_logo() {
return brand_logo;
}
public void setBrand_logo(String brand_logo) {
this.brand_logo = brand_logo;
}
public String getBrand_desc() {
return brand_desc;
}
public void setBrand_desc(String brand_desc) {
this.brand_desc = brand_desc;
}
public String getSite_url() {
return site_url;
}
public void setSite_url(String site_url) {
this.site_url = site_url;
}
public String getSort_order() {
return sort_order;
}
public void setSort_order(String sort_order) {
this.sort_order = sort_order;
}
public String getIs_show() {
return is_show;
}
public void setIs_show(String is_show) {
this.is_show = is_show;
}
public String getStore_id() {
return store_id;
}
public void setStore_id(String store_id) {
this.store_id = store_id;
}
}
<file_sep>/FigoShop/src/main/resources/config/config-hqh.properties
driverClassName=com.mysql.jdbc.Driver
validationQuery=SELECT 1
jdbc_url=jdbc:mysql://172.16.17.32:3306/hqh?useUnicode=true&characterEncoding=UTF-8&zeroDateTimeBehavior=convertToNull
jdbc_username=hqh
jdbc_password=<PASSWORD>^!)@
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/mapper/HaiWebsiteMapper.java
package com.ehais.figoarticle.mapper;
import com.ehais.figoarticle.model.HaiWebsite;
import com.ehais.figoarticle.model.HaiWebsiteExample;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.ResultMap;
import org.apache.ibatis.annotations.Select;
public interface HaiWebsiteMapper {
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_website
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
@Select("select count(*) from hai_website where #{field} = #{value} ")
int unique(@Param("field") String field, @Param("value") String value);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_website
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
@Select("select count(*) from hai_website where #{field} = #{value} and store_id = #{store_id}")
int uniqueStore(@Param("field") String field, @Param("value") String value, @Param("store_id") Integer store_id);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_website
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
long countByExample(HaiWebsiteExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_website
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
int deleteByExample(HaiWebsiteExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_website
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
int insert(HaiWebsite record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_website
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
int insertSelective(HaiWebsite record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_website
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
List<HaiWebsite> selectByExample(HaiWebsiteExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_website
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
int updateByExampleSelective(@Param("record") HaiWebsite record, @Param("example") HaiWebsiteExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_website
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
int updateByExample(@Param("record") HaiWebsite record, @Param("example") HaiWebsiteExample example);
}<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/GoodsattrTOgoods.java
package com.ehais.figoarticle.controller;
import com.ehais.figoarticle.Service.GoodiesAttr;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
/**
* Created by stephen on 5/27/17.
*/
@Controller
public class GoodsattrTOgoods {
@Autowired
private GoodiesAttr goodiesAttr;
@RequestMapping("/GoodsattrTOgoods")
@ResponseBody
public String transfer(){
goodiesAttr.transfer();
return "successed";
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/task/MyTaskAnnotation.java
package com.ehais.figoarticle.task;
import javax.servlet.http.HttpServletRequest;
import org.ehais.util.EHttpClientUtil;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
/**
* 基于注解的定时器 案例
* @author tyler
*/
@Component
public class MyTaskAnnotation {
/**
* [秒] [分] [小时] [日] [月] [周] [年]
* CRON表达式 示例:
"0 0 12 * * ?" 每天中午十二点触发
"0 15 10 ? * *" 每天早上10:15触发
"0 15 10 * * ?" 每天早上10:15触发
"0 15 10 * * ? *" 每天早上10:15触发
"0 15 10 * * ? 2005" 2005年的每天早上10:15触发
"0 * 14 * * ?" 每天从下午2点开始到2点59分每分钟一次触发
"0 0/5 14 * * ?" 每天从下午2点开始到2:55分结束每5分钟一次触发
"0 0/5 14,18 * * ?" 每天的下午2点至2:55和6点至6点55分两个时间段内每5分钟一次触发
"0 0-5 14 * * ?" 每天14:00至14:05每分钟一次触发
"0 10,44 14 ? 3 WED" 三月的每周三的14:10和14:44触发
"0 15 10 ? * MON-FRI" 每个周一、周二、周三、周四、周五的10:15触发
"0 * * * * ?" 每分钟(0点0时0分)触发一次
"0 0 * * * ?" 每小时(0点0时0分)触发一次
*/
@Autowired
private HttpServletRequest request;
/**
* 定时计算。每天凌晨 01:00 执行一次
*/
// @Scheduled(cron = "0 0 1 * * *")
// public void show(){
// System.out.println("Annotation:is show run");
// }
/**
* 心跳更新。启动时执行一次,之后每隔2秒执行一次
*/
// @Scheduled(fixedRate = 1000*2)
// public void print(){
// System.out.println("Annotation:print run");
// }
/**
* 心跳更新。启动时执行一次,之后每隔5秒执行一次
*/
// @Scheduled(cron="0/5 * * * * ? ")
// public void taskCycle(){
// System.out.println("==taskCycle=======================================a");
// }
}
<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/service/HaiNanrcService.java
package com.ehais.hrlucene.service;
public interface HaiNanrcService {
/**
* @描述 检索湖南人才网
* @throws Exception
* @作者 JackChen
* @日期 2016年11月20日
* @返回 void
*/
public void loadHaiNanrc() throws Exception;
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/TheclutcherController.java
package com.ehais.figoarticle.controller;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.ehais.util.Bean2Utils;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.select.Elements;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.model.HaiCategory;
import com.ehais.figoarticle.model.HaiCategoryExample;
import com.ehais.figoarticle.model.HaiGoodsAttr;
import com.ehais.figoarticle.model.HaiGoodsEntity;
import com.ehais.figoarticle.model.HaiGoodsGallery;
import com.ehais.figoarticle.model.HaiGoodsUrl;
import com.ehais.figoarticle.model.HaiGoodsUrlExample;
import com.ehais.figoarticle.model.HaiGoodsWithBLOBs;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
/**
* @author oldbiwang
*
*/
//完成
@Controller
@RequestMapping("/theclutcher")
public class TheclutcherController extends FigoCommonController{
private static String url = "https://www.theclutcher.com";
private int websiteId = 22;
@ResponseBody
@RequestMapping("/brand")
public String brand(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
return "";
}
@ResponseBody
@RequestMapping("/category")
public String category(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
this.category(request, url+"/en-US");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private void category(HttpServletRequest request,String categoryUrl){
String result = "";
try {
/* result = PythonUtil.python("C:/Users/cjc/Desktop/eh-project/FigoShop/getAjaxWeb.py", categoryUrl);
Document doc = Jsoup.parse(result);*/
// result = GetPostTest.sendGet(categoryUrl,null);
// Document doc = Jsoup.parse(result);
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), categoryUrl);
Document doc = Jsoup.parse(result);
//System.out.println(doc);
Element header = doc.getElementsByClass("header").first();
Element blackClearfix = header.select(".black-banner.clearfix").first();
Element nav = blackClearfix.getElementsByClass("nav").first();
Element women = header.select(".women-move-on.sub-menu-bck.cur").first();
Element men = header.select(".men-move-on.sub-menu-bck ").first();
Element babyGirl = header.select(".baby-girl-move-on.sub-menu-bck ").first();
Element babyBoy = header.select(".baby-boy-move-on.sub-menu-bck ").first();
//分类的 List
List<HaiCategory> list = new ArrayList<HaiCategory>();
// System.out.println(nav);
// TODO
Elements mainCategorys = nav.getElementsByTag("li");
String parentHref = "";
//遍历主分类
for(Element mainCategory : mainCategorys) {
System.out.println(mainCategory);
Element a = mainCategory.getElementsByTag("a").first();
System.out.println(a.text());
parentHref = a.attr("href");
if(parentHref.indexOf("http") < 0)
parentHref = url + parentHref;
System.out.println(parentHref);
HaiCategory cat = new HaiCategory();
cat.setCategoryUrl(parentHref);
cat.setCatName(a.text());
cat.setIsShow(true);
cat.setWebsiteId(websiteId);
List<HaiCategory> catList = new ArrayList<HaiCategory>();
if(a.text().equals("WOMAN")) {
Element clearfix = women.getElementsByClass("clearfix").first();
Elements lis = clearfix.getElementsByTag("li");
for(Element li : lis) {
HaiCategory hc = new HaiCategory();
Element la = li.select(">a").first();
if(la.text().equals("DESIGNERS"))
continue;
String href;
href = la.attr("href");
if(href.indexOf("http") < 0)
href = url + href;
hc.setCategoryUrl(href);
hc.setCatName(la.text());
hc.setIsShow(true);
hc.setWebsiteId(websiteId);
catList.add(hc);
}
cat.setChildren(catList);
}
if(a.text().equals("MAN")) {
Element clearfix = men.getElementsByClass("clearfix").first();
Elements lis = clearfix.getElementsByTag("li");
for(Element li : lis) {
HaiCategory hc = new HaiCategory();
Element la = li.select(">a").first();
if(la.text().equals("DESIGNERS"))
continue;
String href;
href = la.attr("href");
if(href.indexOf("http") < 0)
href = url + href;
hc.setCategoryUrl(href);
hc.setCatName(la.text());
hc.setIsShow(true);
hc.setWebsiteId(websiteId);
catList.add(hc);
}
cat.setChildren(catList);
}
if(a.text().equals("BABY GIRL")) {
Element clearfix = babyGirl.getElementsByClass("clearfix").first();
Elements lis = clearfix.getElementsByTag("li");
for(Element li : lis) {
HaiCategory hc = new HaiCategory();
Element la = li.select(">a").first();
if(la.text().equals("DESIGNERS"))
continue;
String href;
href = la.attr("href");
if(href.indexOf("http") < 0)
href = url + href;
hc.setCategoryUrl(href);
hc.setCatName(la.text());
hc.setIsShow(true);
hc.setWebsiteId(websiteId);
catList.add(hc);
}
cat.setChildren(catList);
}
if(a.text().equals("BABY BOY")) {
Element clearfix = babyBoy.getElementsByClass("clearfix").first();
Elements lis = clearfix.getElementsByTag("li");
for(Element li : lis) {
HaiCategory hc = new HaiCategory();
Element la = li.select(">a").first();
if(la.text().equals("DESIGNERS"))
continue;
String href;
href = la.attr("href");
if(href.indexOf("http") < 0)
href = url + href;
hc.setCategoryUrl(href);
hc.setCatName(la.text());
hc.setIsShow(true);
hc.setWebsiteId(websiteId);
catList.add(hc);
}
cat.setChildren(catList);
}
list.add(cat);
}
System.out.println("==========================================");
System.out.println("==========================================");
JSONArray arr = JSONArray.fromObject(list);
System.out.println(arr.toString());
// System.out.println(element.html());
System.out.println("==========================================");
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/category";
// String api = "http://localhost:8087/api/category";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
}
@ResponseBody
@RequestMapping("/url")
public String url(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
c.andCategoryUrlLike(url+"%");
List<HaiCategory> listCategory = haiCategoryMapper.selectByExample(example);
for (HaiCategory haiCategory : listCategory) {
System.out.println(haiCategory.getCategoryUrl());
this.goodsUrl(request, haiCategory.getCategoryUrl(), haiCategory.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsUrl(HttpServletRequest request,String goodsurl,Integer catId){
System.out.println(goodsurl);
String result = "";
try{
// Document doc = Jsoup.connect(goodsurl).timeout(10000).get();
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
Document doc = Jsoup.parse(result);
if(doc == null)
return "";
// System.out.println(doc);
List<String> list = new ArrayList<String>();
// TODO
Element hinder = doc.getElementsByClass("hider").first();
Element clearfixParent = hinder.select(".container.list-box.clearfix").first();
Element clearfix = clearfixParent.getElementsByClass("clearfix").first();
Element products = clearfix.getElementsByTag("ol").first();
Element bottomPager = clearfixParent.getElementsByClass("pager").first();
/*System.out.println(hinder);
System.out.println(clearfixParent);
System.out.println(clearfix);
System.out.println(products);
System.out.println(bottomPager);*/
Elements lis = products.getElementsByClass("product");
// System.out.println(lis);
if(lis == null)
return "";
for(Element li : lis) {
// System.out.println(li);
Element la = li.getElementsByTag("p").first().getElementsByTag("a").first();
// System.out.println(la);
String href = la.attr("href");
if(href.indexOf("http") < 0)
href = url + href;
System.out.println(href);
list.add(href);
}
JSONArray arr = JSONArray.fromObject(list);
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("catId", catId.toString());
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/url";
// String api = "http://localhost:8087/api/url";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
Element currentSpan = bottomPager.getElementsByClass("curr").first();
if(currentSpan != null && currentSpan.text().equals("1") ) {
System.out.println("page 1 start!!!");
Elements pageLis = bottomPager.getElementsByTag("li");
int pageSize = Integer.valueOf(pageLis.get(pageLis.size() - 2).getElementsByTag("a").text());
for(int i = 2; i < pageSize; i++) {
System.out.println("pageSize = " + i);
this.goodsUrl(request, goodsurl + "?currPage=" + i, catId);
}
}
}catch(Exception e){
e.printStackTrace();
}
return result;
}
@ResponseBody
@RequestMapping("/goodsAll")
public String goodsAll(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiGoodsUrlExample example = new HaiGoodsUrlExample();
HaiGoodsUrlExample.Criteria c = example.createCriteria();
c.andGoodsUrlLike(url+"%");
c.andFinishEqualTo(false);
List<HaiGoodsUrl> listGoodsUrl = haiGoodsUrlMapper.selectByExample(example);
for (HaiGoodsUrl haiGoodsUrl : listGoodsUrl) {
goodsModel(request,haiGoodsUrl.getGoodsUrl(),haiGoodsUrl.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
public String goodsModel(HttpServletRequest request , String goodsurl ,Integer catId){
System.out.println(goodsurl);
String result = "";
HaiGoodsEntity entity = new HaiGoodsEntity();
HaiGoodsWithBLOBs goods = new HaiGoodsWithBLOBs();
List<HaiGoodsGallery> goodsGalleryList = new ArrayList<HaiGoodsGallery>();
List<HaiGoodsAttr> goodsAttrList = new ArrayList<HaiGoodsAttr>();
HaiGoodsAttr goodsAttr = new HaiGoodsAttr();
goods.setGoodsUrl(goodsurl);
goods.setCatId(catId);
goods.setWebsiteId(websiteId);
try{
// Document doc = Jsoup.connect(goodsurl).timeout(10000).get();
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
Document doc = Jsoup.parse(result);
if(doc == null)
return "";
Element boxClearfix = doc.select(".box.clearfix").first();
Element col = boxClearfix.getElementsByClass("col").first();
Element colDetails = boxClearfix.select(".box.clearfix").first();
Element info = colDetails.getElementsByClass("info").first();
Element detail = info.getElementsByTag("p").first();
goods.setGoodsDesc(detail.toString());
//设置商品名字
Element productName = colDetails.getElementsByTag("h1").first().getElementsByTag("a").first();
System.out.println(productName.text());
goods.setGoodsName(productName.text());
//设置价格
Integer pri;
Element priceBox = colDetails.getElementsByClass("price-box").first();
if(priceBox == null)
return "";
Element price = priceBox.getElementsByClass("promo").first();
if(price == null)
return "";
int index1 = price.text().indexOf(' ');
int index2 = price.text().indexOf('.');
int index3 = price.text().indexOf(',');
if(index1 < 0 || index2 < 0 || index3 < 0)
return "";
pri = Integer.parseInt(price.text().substring(index1 + 1 , index2)) * 1000 + Integer.parseInt(price.text().substring(index2 + 1 , index3));
System.out.println(pri);
goods.setShopPrice(pri);
String currency = price.text().substring(0,4);
System.out.println(currency);
goods.setCurrency(currency);
//设置size
Element sizeandcolor = colDetails.getElementsByClass("size-and-color").first();
Element sizes = sizeandcolor.getElementsByClass("sizes").first();
Elements labels = sizes.getElementsByTag("label");
for(Element label : labels) {
goodsAttr = new HaiGoodsAttr();
goodsAttr.setAttrValue(label.text());
goodsAttr.setAttrType("size");
goodsAttr.setAttrPrice(pri.toString());
goodsAttrList.add(goodsAttr);
}
//设置color
Element description = info.getElementsByTag("p").first();
String color="";
String colorstr="";
System.out.println(description.ownText());
int index4 = description.ownText().indexOf("Color: ");
if(index4 < 0) {
int index6 = description.ownText().indexOf("Colour: ");
System.out.println(index6);
if(index6 < 0) {
int index7 = description.ownText().indexOf("Color. ");
System.out.println(index7);
if(index7 < 0)
return "";
colorstr = description.ownText().substring(index7 + 7);
System.out.println(colorstr);
int index5 = colorstr.indexOf(' ');
System.out.println(index5);
if(index5 < 0)
return "";
color = colorstr.substring(0,index5);
}
else {
colorstr = description.ownText().substring(index6 + 8);
System.out.println(colorstr);
int index5 = colorstr.indexOf(' ');
System.out.println(index5);
if(index5 < 0)
return "";
color = colorstr.substring(0,index5);
}
}else {
colorstr = description.ownText().substring(index4 + 7);
System.out.println(colorstr);
int index5 = colorstr.indexOf(' ');
System.out.println(index5);
if(index5 < 0)
return "";
color = colorstr.substring(0,index5);
}
HaiGoodsAttr goodsColor = new HaiGoodsAttr();
goodsColor.setAttrValue(color);
goodsColor.setAttrType("color");
goodsColor.setAttrPrice(pri.toString());
goodsAttrList.add(goodsColor);
//设置图片
Element big = col.getElementsByClass("photo-big").first();
Elements divs = big.select(">div");
for(Element dataThumb : divs) {
Element img = dataThumb.getElementsByTag("img").first();
HaiGoodsGallery gallery = new HaiGoodsGallery();
gallery.setThumbUrl(dataThumb.attr("data-thumb"));
gallery.setImgUrl(img.attr("src"));
gallery.setImgOriginal(img.attr("src"));
goodsGalleryList.add(gallery);
}
if(goodsGalleryList.size() > 0) {
HaiGoodsGallery gallery = goodsGalleryList.get(0);
goods.setGoodsThumb(gallery.getThumbUrl());
goods.setGoodsImg(gallery.getImgUrl());
goods.setOriginalImg(gallery.getImgOriginal());
}
entity.setGoods(goods);
entity.setGoodsAttrList(goodsAttrList);
entity.setGoodsGalleryList(goodsGalleryList);
JSONObject jsonObject = JSONObject.fromObject(entity);
System.out.println(jsonObject.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", jsonObject.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/goodsAttr";
// String api = "http://localhost:8087/api/goodsAttr";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
//单商品的地址请求
@ResponseBody
@RequestMapping("/getGoodsUrl")
public String getGoodsUrl(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "catId", required = true) Integer catId,
@RequestParam(value = "goodsurl", required = true) String goodsurl
){
try{
return goodsModel(request,goodsurl,catId);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
public static void main(String[] args) throws Exception {
String goodsurl = "https://www.net-a-porter.com/cn/zh/d/Shop/Lingerie/All?cm_sp=topnav-_-clothing-_-lingerie";
DemoController ac = new DemoController();
// ac.goodsModel(url,1);
// ac.goodsUrl(null, goodsurl, 1);
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/HaiCategoryExample.java
package com.ehais.figoarticle.model;
import java.util.ArrayList;
import java.util.List;
import org.ehais.tools.CriteriaObject;
public class HaiCategoryExample {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected String orderByClause;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected boolean distinct;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected List<Criteria> oredCriteria;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected int limitStart = -1;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected int limitEnd = -1;
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public HaiCategoryExample() {
oredCriteria = new ArrayList<Criteria>();
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public String getOrderByClause() {
return orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public boolean isDistinct() {
return distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setLimitStart(int limitStart) {
this.limitStart=limitStart;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public int getLimitStart() {
return limitStart;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public void setLimitEnd(int limitEnd) {
this.limitEnd=limitEnd;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public int getLimitEnd() {
return limitEnd;
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andCatIdIsNull() {
addCriterion("cat_id is null");
return (Criteria) this;
}
public Criteria andCatIdIsNotNull() {
addCriterion("cat_id is not null");
return (Criteria) this;
}
public Criteria andCatIdEqualTo(Integer value) {
addCriterion("cat_id =", value, "catId");
return (Criteria) this;
}
public Criteria andCatIdNotEqualTo(Integer value) {
addCriterion("cat_id <>", value, "catId");
return (Criteria) this;
}
public Criteria andCatIdGreaterThan(Integer value) {
addCriterion("cat_id >", value, "catId");
return (Criteria) this;
}
public Criteria andCatIdGreaterThanOrEqualTo(Integer value) {
addCriterion("cat_id >=", value, "catId");
return (Criteria) this;
}
public Criteria andCatIdLessThan(Integer value) {
addCriterion("cat_id <", value, "catId");
return (Criteria) this;
}
public Criteria andCatIdLessThanOrEqualTo(Integer value) {
addCriterion("cat_id <=", value, "catId");
return (Criteria) this;
}
public Criteria andCatIdIn(List<Integer> values) {
addCriterion("cat_id in", values, "catId");
return (Criteria) this;
}
public Criteria andCatIdNotIn(List<Integer> values) {
addCriterion("cat_id not in", values, "catId");
return (Criteria) this;
}
public Criteria andCatIdBetween(Integer value1, Integer value2) {
addCriterion("cat_id between", value1, value2, "catId");
return (Criteria) this;
}
public Criteria andCatIdNotBetween(Integer value1, Integer value2) {
addCriterion("cat_id not between", value1, value2, "catId");
return (Criteria) this;
}
public Criteria andCatNameIsNull() {
addCriterion("cat_name is null");
return (Criteria) this;
}
public Criteria andCatNameIsNotNull() {
addCriterion("cat_name is not null");
return (Criteria) this;
}
public Criteria andCatNameEqualTo(String value) {
addCriterion("cat_name =", value, "catName");
return (Criteria) this;
}
public Criteria andCatNameNotEqualTo(String value) {
addCriterion("cat_name <>", value, "catName");
return (Criteria) this;
}
public Criteria andCatNameGreaterThan(String value) {
addCriterion("cat_name >", value, "catName");
return (Criteria) this;
}
public Criteria andCatNameGreaterThanOrEqualTo(String value) {
addCriterion("cat_name >=", value, "catName");
return (Criteria) this;
}
public Criteria andCatNameLessThan(String value) {
addCriterion("cat_name <", value, "catName");
return (Criteria) this;
}
public Criteria andCatNameLessThanOrEqualTo(String value) {
addCriterion("cat_name <=", value, "catName");
return (Criteria) this;
}
public Criteria andCatNameLike(String value) {
addCriterion("cat_name like", value, "catName");
return (Criteria) this;
}
public Criteria andCatNameNotLike(String value) {
addCriterion("cat_name not like", value, "catName");
return (Criteria) this;
}
public Criteria andCatNameIn(List<String> values) {
addCriterion("cat_name in", values, "catName");
return (Criteria) this;
}
public Criteria andCatNameNotIn(List<String> values) {
addCriterion("cat_name not in", values, "catName");
return (Criteria) this;
}
public Criteria andCatNameBetween(String value1, String value2) {
addCriterion("cat_name between", value1, value2, "catName");
return (Criteria) this;
}
public Criteria andCatNameNotBetween(String value1, String value2) {
addCriterion("cat_name not between", value1, value2, "catName");
return (Criteria) this;
}
public Criteria andKeywordsIsNull() {
addCriterion("keywords is null");
return (Criteria) this;
}
public Criteria andKeywordsIsNotNull() {
addCriterion("keywords is not null");
return (Criteria) this;
}
public Criteria andKeywordsEqualTo(String value) {
addCriterion("keywords =", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsNotEqualTo(String value) {
addCriterion("keywords <>", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsGreaterThan(String value) {
addCriterion("keywords >", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsGreaterThanOrEqualTo(String value) {
addCriterion("keywords >=", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsLessThan(String value) {
addCriterion("keywords <", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsLessThanOrEqualTo(String value) {
addCriterion("keywords <=", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsLike(String value) {
addCriterion("keywords like", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsNotLike(String value) {
addCriterion("keywords not like", value, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsIn(List<String> values) {
addCriterion("keywords in", values, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsNotIn(List<String> values) {
addCriterion("keywords not in", values, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsBetween(String value1, String value2) {
addCriterion("keywords between", value1, value2, "keywords");
return (Criteria) this;
}
public Criteria andKeywordsNotBetween(String value1, String value2) {
addCriterion("keywords not between", value1, value2, "keywords");
return (Criteria) this;
}
public Criteria andCatDescIsNull() {
addCriterion("cat_desc is null");
return (Criteria) this;
}
public Criteria andCatDescIsNotNull() {
addCriterion("cat_desc is not null");
return (Criteria) this;
}
public Criteria andCatDescEqualTo(String value) {
addCriterion("cat_desc =", value, "catDesc");
return (Criteria) this;
}
public Criteria andCatDescNotEqualTo(String value) {
addCriterion("cat_desc <>", value, "catDesc");
return (Criteria) this;
}
public Criteria andCatDescGreaterThan(String value) {
addCriterion("cat_desc >", value, "catDesc");
return (Criteria) this;
}
public Criteria andCatDescGreaterThanOrEqualTo(String value) {
addCriterion("cat_desc >=", value, "catDesc");
return (Criteria) this;
}
public Criteria andCatDescLessThan(String value) {
addCriterion("cat_desc <", value, "catDesc");
return (Criteria) this;
}
public Criteria andCatDescLessThanOrEqualTo(String value) {
addCriterion("cat_desc <=", value, "catDesc");
return (Criteria) this;
}
public Criteria andCatDescLike(String value) {
addCriterion("cat_desc like", value, "catDesc");
return (Criteria) this;
}
public Criteria andCatDescNotLike(String value) {
addCriterion("cat_desc not like", value, "catDesc");
return (Criteria) this;
}
public Criteria andCatDescIn(List<String> values) {
addCriterion("cat_desc in", values, "catDesc");
return (Criteria) this;
}
public Criteria andCatDescNotIn(List<String> values) {
addCriterion("cat_desc not in", values, "catDesc");
return (Criteria) this;
}
public Criteria andCatDescBetween(String value1, String value2) {
addCriterion("cat_desc between", value1, value2, "catDesc");
return (Criteria) this;
}
public Criteria andCatDescNotBetween(String value1, String value2) {
addCriterion("cat_desc not between", value1, value2, "catDesc");
return (Criteria) this;
}
public Criteria andParentIdIsNull() {
addCriterion("parent_id is null");
return (Criteria) this;
}
public Criteria andParentIdIsNotNull() {
addCriterion("parent_id is not null");
return (Criteria) this;
}
public Criteria andParentIdEqualTo(Integer value) {
addCriterion("parent_id =", value, "parentId");
return (Criteria) this;
}
public Criteria andParentIdNotEqualTo(Integer value) {
addCriterion("parent_id <>", value, "parentId");
return (Criteria) this;
}
public Criteria andParentIdGreaterThan(Integer value) {
addCriterion("parent_id >", value, "parentId");
return (Criteria) this;
}
public Criteria andParentIdGreaterThanOrEqualTo(Integer value) {
addCriterion("parent_id >=", value, "parentId");
return (Criteria) this;
}
public Criteria andParentIdLessThan(Integer value) {
addCriterion("parent_id <", value, "parentId");
return (Criteria) this;
}
public Criteria andParentIdLessThanOrEqualTo(Integer value) {
addCriterion("parent_id <=", value, "parentId");
return (Criteria) this;
}
public Criteria andParentIdIn(List<Integer> values) {
addCriterion("parent_id in", values, "parentId");
return (Criteria) this;
}
public Criteria andParentIdNotIn(List<Integer> values) {
addCriterion("parent_id not in", values, "parentId");
return (Criteria) this;
}
public Criteria andParentIdBetween(Integer value1, Integer value2) {
addCriterion("parent_id between", value1, value2, "parentId");
return (Criteria) this;
}
public Criteria andParentIdNotBetween(Integer value1, Integer value2) {
addCriterion("parent_id not between", value1, value2, "parentId");
return (Criteria) this;
}
public Criteria andSortOrderIsNull() {
addCriterion("sort_order is null");
return (Criteria) this;
}
public Criteria andSortOrderIsNotNull() {
addCriterion("sort_order is not null");
return (Criteria) this;
}
public Criteria andSortOrderEqualTo(Short value) {
addCriterion("sort_order =", value, "sortOrder");
return (Criteria) this;
}
public Criteria andSortOrderNotEqualTo(Short value) {
addCriterion("sort_order <>", value, "sortOrder");
return (Criteria) this;
}
public Criteria andSortOrderGreaterThan(Short value) {
addCriterion("sort_order >", value, "sortOrder");
return (Criteria) this;
}
public Criteria andSortOrderGreaterThanOrEqualTo(Short value) {
addCriterion("sort_order >=", value, "sortOrder");
return (Criteria) this;
}
public Criteria andSortOrderLessThan(Short value) {
addCriterion("sort_order <", value, "sortOrder");
return (Criteria) this;
}
public Criteria andSortOrderLessThanOrEqualTo(Short value) {
addCriterion("sort_order <=", value, "sortOrder");
return (Criteria) this;
}
public Criteria andSortOrderIn(List<Short> values) {
addCriterion("sort_order in", values, "sortOrder");
return (Criteria) this;
}
public Criteria andSortOrderNotIn(List<Short> values) {
addCriterion("sort_order not in", values, "sortOrder");
return (Criteria) this;
}
public Criteria andSortOrderBetween(Short value1, Short value2) {
addCriterion("sort_order between", value1, value2, "sortOrder");
return (Criteria) this;
}
public Criteria andSortOrderNotBetween(Short value1, Short value2) {
addCriterion("sort_order not between", value1, value2, "sortOrder");
return (Criteria) this;
}
public Criteria andIsShowIsNull() {
addCriterion("is_show is null");
return (Criteria) this;
}
public Criteria andIsShowIsNotNull() {
addCriterion("is_show is not null");
return (Criteria) this;
}
public Criteria andIsShowEqualTo(Boolean value) {
addCriterion("is_show =", value, "isShow");
return (Criteria) this;
}
public Criteria andIsShowNotEqualTo(Boolean value) {
addCriterion("is_show <>", value, "isShow");
return (Criteria) this;
}
public Criteria andIsShowGreaterThan(Boolean value) {
addCriterion("is_show >", value, "isShow");
return (Criteria) this;
}
public Criteria andIsShowGreaterThanOrEqualTo(Boolean value) {
addCriterion("is_show >=", value, "isShow");
return (Criteria) this;
}
public Criteria andIsShowLessThan(Boolean value) {
addCriterion("is_show <", value, "isShow");
return (Criteria) this;
}
public Criteria andIsShowLessThanOrEqualTo(Boolean value) {
addCriterion("is_show <=", value, "isShow");
return (Criteria) this;
}
public Criteria andIsShowIn(List<Boolean> values) {
addCriterion("is_show in", values, "isShow");
return (Criteria) this;
}
public Criteria andIsShowNotIn(List<Boolean> values) {
addCriterion("is_show not in", values, "isShow");
return (Criteria) this;
}
public Criteria andIsShowBetween(Boolean value1, Boolean value2) {
addCriterion("is_show between", value1, value2, "isShow");
return (Criteria) this;
}
public Criteria andIsShowNotBetween(Boolean value1, Boolean value2) {
addCriterion("is_show not between", value1, value2, "isShow");
return (Criteria) this;
}
public Criteria andCategoryUrlIsNull() {
addCriterion("category_url is null");
return (Criteria) this;
}
public Criteria andCategoryUrlIsNotNull() {
addCriterion("category_url is not null");
return (Criteria) this;
}
public Criteria andCategoryUrlEqualTo(String value) {
addCriterion("category_url =", value, "categoryUrl");
return (Criteria) this;
}
public Criteria andCategoryUrlNotEqualTo(String value) {
addCriterion("category_url <>", value, "categoryUrl");
return (Criteria) this;
}
public Criteria andCategoryUrlGreaterThan(String value) {
addCriterion("category_url >", value, "categoryUrl");
return (Criteria) this;
}
public Criteria andCategoryUrlGreaterThanOrEqualTo(String value) {
addCriterion("category_url >=", value, "categoryUrl");
return (Criteria) this;
}
public Criteria andCategoryUrlLessThan(String value) {
addCriterion("category_url <", value, "categoryUrl");
return (Criteria) this;
}
public Criteria andCategoryUrlLessThanOrEqualTo(String value) {
addCriterion("category_url <=", value, "categoryUrl");
return (Criteria) this;
}
public Criteria andCategoryUrlLike(String value) {
addCriterion("category_url like", value, "categoryUrl");
return (Criteria) this;
}
public Criteria andCategoryUrlNotLike(String value) {
addCriterion("category_url not like", value, "categoryUrl");
return (Criteria) this;
}
public Criteria andCategoryUrlIn(List<String> values) {
addCriterion("category_url in", values, "categoryUrl");
return (Criteria) this;
}
public Criteria andCategoryUrlNotIn(List<String> values) {
addCriterion("category_url not in", values, "categoryUrl");
return (Criteria) this;
}
public Criteria andCategoryUrlBetween(String value1, String value2) {
addCriterion("category_url between", value1, value2, "categoryUrl");
return (Criteria) this;
}
public Criteria andCategoryUrlNotBetween(String value1, String value2) {
addCriterion("category_url not between", value1, value2, "categoryUrl");
return (Criteria) this;
}
public Criteria andCidIsNull() {
addCriterion("cid is null");
return (Criteria) this;
}
public Criteria andCidIsNotNull() {
addCriterion("cid is not null");
return (Criteria) this;
}
public Criteria andCidEqualTo(Integer value) {
addCriterion("cid =", value, "cid");
return (Criteria) this;
}
public Criteria andCidNotEqualTo(Integer value) {
addCriterion("cid <>", value, "cid");
return (Criteria) this;
}
public Criteria andCidGreaterThan(Integer value) {
addCriterion("cid >", value, "cid");
return (Criteria) this;
}
public Criteria andCidGreaterThanOrEqualTo(Integer value) {
addCriterion("cid >=", value, "cid");
return (Criteria) this;
}
public Criteria andCidLessThan(Integer value) {
addCriterion("cid <", value, "cid");
return (Criteria) this;
}
public Criteria andCidLessThanOrEqualTo(Integer value) {
addCriterion("cid <=", value, "cid");
return (Criteria) this;
}
public Criteria andCidIn(List<Integer> values) {
addCriterion("cid in", values, "cid");
return (Criteria) this;
}
public Criteria andCidNotIn(List<Integer> values) {
addCriterion("cid not in", values, "cid");
return (Criteria) this;
}
public Criteria andCidBetween(Integer value1, Integer value2) {
addCriterion("cid between", value1, value2, "cid");
return (Criteria) this;
}
public Criteria andCidNotBetween(Integer value1, Integer value2) {
addCriterion("cid not between", value1, value2, "cid");
return (Criteria) this;
}
public Criteria andCreatedIsNull() {
addCriterion("created is null");
return (Criteria) this;
}
public Criteria andCreatedIsNotNull() {
addCriterion("created is not null");
return (Criteria) this;
}
public Criteria andCreatedEqualTo(Integer value) {
addCriterion("created =", value, "created");
return (Criteria) this;
}
public Criteria andCreatedNotEqualTo(Integer value) {
addCriterion("created <>", value, "created");
return (Criteria) this;
}
public Criteria andCreatedGreaterThan(Integer value) {
addCriterion("created >", value, "created");
return (Criteria) this;
}
public Criteria andCreatedGreaterThanOrEqualTo(Integer value) {
addCriterion("created >=", value, "created");
return (Criteria) this;
}
public Criteria andCreatedLessThan(Integer value) {
addCriterion("created <", value, "created");
return (Criteria) this;
}
public Criteria andCreatedLessThanOrEqualTo(Integer value) {
addCriterion("created <=", value, "created");
return (Criteria) this;
}
public Criteria andCreatedIn(List<Integer> values) {
addCriterion("created in", values, "created");
return (Criteria) this;
}
public Criteria andCreatedNotIn(List<Integer> values) {
addCriterion("created not in", values, "created");
return (Criteria) this;
}
public Criteria andCreatedBetween(Integer value1, Integer value2) {
addCriterion("created between", value1, value2, "created");
return (Criteria) this;
}
public Criteria andCreatedNotBetween(Integer value1, Integer value2) {
addCriterion("created not between", value1, value2, "created");
return (Criteria) this;
}
public Criteria andUpdatedIsNull() {
addCriterion("updated is null");
return (Criteria) this;
}
public Criteria andUpdatedIsNotNull() {
addCriterion("updated is not null");
return (Criteria) this;
}
public Criteria andUpdatedEqualTo(Integer value) {
addCriterion("updated =", value, "updated");
return (Criteria) this;
}
public Criteria andUpdatedNotEqualTo(Integer value) {
addCriterion("updated <>", value, "updated");
return (Criteria) this;
}
public Criteria andUpdatedGreaterThan(Integer value) {
addCriterion("updated >", value, "updated");
return (Criteria) this;
}
public Criteria andUpdatedGreaterThanOrEqualTo(Integer value) {
addCriterion("updated >=", value, "updated");
return (Criteria) this;
}
public Criteria andUpdatedLessThan(Integer value) {
addCriterion("updated <", value, "updated");
return (Criteria) this;
}
public Criteria andUpdatedLessThanOrEqualTo(Integer value) {
addCriterion("updated <=", value, "updated");
return (Criteria) this;
}
public Criteria andUpdatedIn(List<Integer> values) {
addCriterion("updated in", values, "updated");
return (Criteria) this;
}
public Criteria andUpdatedNotIn(List<Integer> values) {
addCriterion("updated not in", values, "updated");
return (Criteria) this;
}
public Criteria andUpdatedBetween(Integer value1, Integer value2) {
addCriterion("updated between", value1, value2, "updated");
return (Criteria) this;
}
public Criteria andUpdatedNotBetween(Integer value1, Integer value2) {
addCriterion("updated not between", value1, value2, "updated");
return (Criteria) this;
}
public Criteria andDeletedIsNull() {
addCriterion("deleted is null");
return (Criteria) this;
}
public Criteria andDeletedIsNotNull() {
addCriterion("deleted is not null");
return (Criteria) this;
}
public Criteria andDeletedEqualTo(Integer value) {
addCriterion("deleted =", value, "deleted");
return (Criteria) this;
}
public Criteria andDeletedNotEqualTo(Integer value) {
addCriterion("deleted <>", value, "deleted");
return (Criteria) this;
}
public Criteria andDeletedGreaterThan(Integer value) {
addCriterion("deleted >", value, "deleted");
return (Criteria) this;
}
public Criteria andDeletedGreaterThanOrEqualTo(Integer value) {
addCriterion("deleted >=", value, "deleted");
return (Criteria) this;
}
public Criteria andDeletedLessThan(Integer value) {
addCriterion("deleted <", value, "deleted");
return (Criteria) this;
}
public Criteria andDeletedLessThanOrEqualTo(Integer value) {
addCriterion("deleted <=", value, "deleted");
return (Criteria) this;
}
public Criteria andDeletedIn(List<Integer> values) {
addCriterion("deleted in", values, "deleted");
return (Criteria) this;
}
public Criteria andDeletedNotIn(List<Integer> values) {
addCriterion("deleted not in", values, "deleted");
return (Criteria) this;
}
public Criteria andDeletedBetween(Integer value1, Integer value2) {
addCriterion("deleted between", value1, value2, "deleted");
return (Criteria) this;
}
public Criteria andDeletedNotBetween(Integer value1, Integer value2) {
addCriterion("deleted not between", value1, value2, "deleted");
return (Criteria) this;
}
public Criteria andWebsiteIdIsNull() {
addCriterion("website_id is null");
return (Criteria) this;
}
public Criteria andWebsiteIdIsNotNull() {
addCriterion("website_id is not null");
return (Criteria) this;
}
public Criteria andWebsiteIdEqualTo(Integer value) {
addCriterion("website_id =", value, "websiteId");
return (Criteria) this;
}
public Criteria andWebsiteIdNotEqualTo(Integer value) {
addCriterion("website_id <>", value, "websiteId");
return (Criteria) this;
}
public Criteria andWebsiteIdGreaterThan(Integer value) {
addCriterion("website_id >", value, "websiteId");
return (Criteria) this;
}
public Criteria andWebsiteIdGreaterThanOrEqualTo(Integer value) {
addCriterion("website_id >=", value, "websiteId");
return (Criteria) this;
}
public Criteria andWebsiteIdLessThan(Integer value) {
addCriterion("website_id <", value, "websiteId");
return (Criteria) this;
}
public Criteria andWebsiteIdLessThanOrEqualTo(Integer value) {
addCriterion("website_id <=", value, "websiteId");
return (Criteria) this;
}
public Criteria andWebsiteIdIn(List<Integer> values) {
addCriterion("website_id in", values, "websiteId");
return (Criteria) this;
}
public Criteria andWebsiteIdNotIn(List<Integer> values) {
addCriterion("website_id not in", values, "websiteId");
return (Criteria) this;
}
public Criteria andWebsiteIdBetween(Integer value1, Integer value2) {
addCriterion("website_id between", value1, value2, "websiteId");
return (Criteria) this;
}
public Criteria andWebsiteIdNotBetween(Integer value1, Integer value2) {
addCriterion("website_id not between", value1, value2, "websiteId");
return (Criteria) this;
}
public Criteria andCalculateIsNull() {
addCriterion("calculate is null");
return (Criteria) this;
}
public Criteria andCalculateIsNotNull() {
addCriterion("calculate is not null");
return (Criteria) this;
}
public Criteria andCalculateEqualTo(String value) {
addCriterion("calculate =", value, "calculate");
return (Criteria) this;
}
public Criteria andCalculateNotEqualTo(String value) {
addCriterion("calculate <>", value, "calculate");
return (Criteria) this;
}
public Criteria andCalculateGreaterThan(String value) {
addCriterion("calculate >", value, "calculate");
return (Criteria) this;
}
public Criteria andCalculateGreaterThanOrEqualTo(String value) {
addCriterion("calculate >=", value, "calculate");
return (Criteria) this;
}
public Criteria andCalculateLessThan(String value) {
addCriterion("calculate <", value, "calculate");
return (Criteria) this;
}
public Criteria andCalculateLessThanOrEqualTo(String value) {
addCriterion("calculate <=", value, "calculate");
return (Criteria) this;
}
public Criteria andCalculateLike(String value) {
addCriterion("calculate like", value, "calculate");
return (Criteria) this;
}
public Criteria andCalculateNotLike(String value) {
addCriterion("calculate not like", value, "calculate");
return (Criteria) this;
}
public Criteria andCalculateIn(List<String> values) {
addCriterion("calculate in", values, "calculate");
return (Criteria) this;
}
public Criteria andCalculateNotIn(List<String> values) {
addCriterion("calculate not in", values, "calculate");
return (Criteria) this;
}
public Criteria andCalculateBetween(String value1, String value2) {
addCriterion("calculate between", value1, value2, "calculate");
return (Criteria) this;
}
public Criteria andCalculateNotBetween(String value1, String value2) {
addCriterion("calculate not between", value1, value2, "calculate");
return (Criteria) this;
}
public Criteria andSpiderSourceIsNull() {
addCriterion("spider_source is null");
return (Criteria) this;
}
public Criteria andSpiderSourceIsNotNull() {
addCriterion("spider_source is not null");
return (Criteria) this;
}
public Criteria andSpiderSourceEqualTo(String value) {
addCriterion("spider_source =", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceNotEqualTo(String value) {
addCriterion("spider_source <>", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceGreaterThan(String value) {
addCriterion("spider_source >", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceGreaterThanOrEqualTo(String value) {
addCriterion("spider_source >=", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceLessThan(String value) {
addCriterion("spider_source <", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceLessThanOrEqualTo(String value) {
addCriterion("spider_source <=", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceLike(String value) {
addCriterion("spider_source like", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceNotLike(String value) {
addCriterion("spider_source not like", value, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceIn(List<String> values) {
addCriterion("spider_source in", values, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceNotIn(List<String> values) {
addCriterion("spider_source not in", values, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceBetween(String value1, String value2) {
addCriterion("spider_source between", value1, value2, "spiderSource");
return (Criteria) this;
}
public Criteria andSpiderSourceNotBetween(String value1, String value2) {
addCriterion("spider_source not between", value1, value2, "spiderSource");
return (Criteria) this;
}
public Criteria andCatNameLikeInsensitive(String value) {
addCriterion("upper(cat_name) like", value.toUpperCase(), "catName");
return (Criteria) this;
}
public Criteria andKeywordsLikeInsensitive(String value) {
addCriterion("upper(keywords) like", value.toUpperCase(), "keywords");
return (Criteria) this;
}
public Criteria andCatDescLikeInsensitive(String value) {
addCriterion("upper(cat_desc) like", value.toUpperCase(), "catDesc");
return (Criteria) this;
}
public Criteria andCategoryUrlLikeInsensitive(String value) {
addCriterion("upper(category_url) like", value.toUpperCase(), "categoryUrl");
return (Criteria) this;
}
public Criteria andCalculateLikeInsensitive(String value) {
addCriterion("upper(calculate) like", value.toUpperCase(), "calculate");
return (Criteria) this;
}
public Criteria andSpiderSourceLikeInsensitive(String value) {
addCriterion("upper(spider_source) like", value.toUpperCase(), "spiderSource");
return (Criteria) this;
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table hai_category
*
* @mbg.generated do_not_delete_during_merge Sun Nov 26 11:43:10 CST 2017
*/
public static class Criteria extends GeneratedCriteria {
protected Criteria() {
super();
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table hai_category
*
* @mbg.generated Sun Nov 26 11:43:10 CST 2017
*/
public static class Criterion {
private String condition;
private Object value;
private Object secondValue;
private boolean noValue;
private boolean singleValue;
private boolean betweenValue;
private boolean listValue;
private String typeHandler;
public String getCondition() {
return condition;
}
public Object getValue() {
return value;
}
public Object getSecondValue() {
return secondValue;
}
public boolean isNoValue() {
return noValue;
}
public boolean isSingleValue() {
return singleValue;
}
public boolean isBetweenValue() {
return betweenValue;
}
public boolean isListValue() {
return listValue;
}
public String getTypeHandler() {
return typeHandler;
}
protected Criterion(String condition) {
super();
this.condition = condition;
this.typeHandler = null;
this.noValue = true;
}
protected Criterion(String condition, Object value, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.typeHandler = typeHandler;
if (value instanceof List<?>) {
this.listValue = true;
} else {
this.singleValue = true;
}
}
protected Criterion(String condition, Object value) {
this(condition, value, null);
}
protected Criterion(String condition, Object value, Object secondValue, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.secondValue = secondValue;
this.typeHandler = typeHandler;
this.betweenValue = true;
}
protected Criterion(String condition, Object value, Object secondValue) {
this(condition, value, secondValue, null);
}
}
}<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/service/FjrcService.java
package com.ehais.hrlucene.service;
public interface FjrcService {
/**
* @描述 检索海峡人才网
* @throws Exception
* @作者 Stephen-Zhang referred from lgj
* @日期 2016年11月20日
* @返回 void
*/
public void LoadFjrc() throws Exception ;
}
<file_sep>/school-weixin/pom.xml
<?xml version="1.0"?>
<project xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd" xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>com.ehais</groupId>
<artifactId>ehproject</artifactId>
<version>0.0.1-SNAPSHOT</version>
</parent>
<groupId>com.ehais</groupId>
<artifactId>school-weixin</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>school-weixin</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<controller.package>com.ehais.school.controller,com.ehais.school.webservice,org.ehais.weixin.controller,org.ehais.controller,com.ehais.schoolweixin.controller</controller.package>
<component.package>com.ehais.school.service,com.ehais.hrlucene.service,org.ehais.weixin.service,org.ehais.service,com.ehais.schoolweixin.service</component.package>
<mapper.xml>classpath*:mapper/**/*.xml</mapper.xml>
<model.package>com.ehais.school.model,com.ehais.hrlucene.model,org.ehais.weixin.model,org.ehais.model,com.ehais.schoolweixin.model</model.package>
<mapper.package>com.ehais.school.mapper,com.ehais.hrlucene.mapper,org.ehais.weixin.mapper,org.ehais.mapper,com.ehais.schoolweixin.mapper</mapper.package>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
<dependency>
<groupId>org.freemarker</groupId>
<artifactId>freemarker</artifactId>
<version>2.3.23</version>
</dependency>
<dependency>
<groupId>com.ehais</groupId>
<artifactId>eh-common</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>com.ehais</groupId>
<artifactId>eh-weixin</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>com.ehais</groupId>
<artifactId>eHR-Lucene</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
<build>
<finalName>school-weixin</finalName>
<resources>
<resource>
<directory>src/main/resources</directory>
<filtering>true</filtering>
</resource>
</resources>
<plugins>
<plugin>
<groupId>org.mybatis.generator</groupId>
<artifactId>mybatis-generator-maven-plugin</artifactId>
<version>1.3.2</version>
<configuration>
<verbose>true</verbose>
<overwrite>true</overwrite>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>${maven_compiler_ver}</version>
<configuration>
<source>1.7</source>
<target>1.7</target>
<encoding>${project.build.sourceEncoding}</encoding>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-resources-plugin</artifactId>
<version>${maven_resources_ver}</version>
<configuration>
<encoding>${project.build.sourceEncoding}</encoding>
</configuration>
</plugin>
<!-- eclipse -X tomcat7:run -->
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.1</version>
<configuration>
<path>/</path>
<uriEncoding>UTF-8</uriEncoding>
<port>8080</port>
<java.util.logging.manager>org.apache.juli.ClassLoaderLogManager</java.util.logging.manager>
<mode>context</mode>
<contextFile>${basedir}/../src/main/resources/context.xml</contextFile>
</configuration>
</plugin>
<!-- clean package jboss-as:deploy -->
<plugin>
<groupId>org.jboss.as.plugins</groupId>
<artifactId>jboss-as-maven-plugin</artifactId>
<version>7.3.Final</version>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>2.0.2</version>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-resources-plugin</artifactId>
<configuration>
<encoding>${project.build.sourceEncoding}</encoding>
</configuration>
<executions>
<execution>
<id>copy-resources-config-properties</id>
<phase>validate</phase>
<goals>
<goal>copy-resources</goal>
</goals>
<configuration>
<outputDirectory>${basedir}/target/classes/META-INF/config/</outputDirectory>
<resources>
<resource>
<directory>${basedir}/../src/main/resources/config/</directory>
<includes>
<include>**/*.properties</include>
</includes>
</resource>
</resources>
</configuration>
</execution>
<execution>
<id>copy-xmls</id>
<phase>process-sources</phase>
<goals>
<goal>copy-resources</goal>
</goals>
<configuration>
<outputDirectory>${basedir}/target/classes/META-INF/resources/ehais/</outputDirectory>
<resources>
<resource>
<directory>${basedir}/../eh-common/src/main/ehais-resources/ehais/</directory>
</resource>
</resources>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
<packaging>war</packaging>
</project>
<file_sep>/reptileV1/src/main/java/com/ehais/reptile/Reptile.java
package com.ehais.reptile;
import java.util.List;
import java.util.Map;
import org.ehais.tools.ReturnObject;
import org.jsoup.nodes.Element;
import org.junit.Test;
public interface Reptile {
@Test
public void list();
public ReturnObject<Object> product_list(String url) throws Exception;
@Test
public void info();
public ReturnObject<Object> product_info(String url) throws Exception;
public List<Map<String,String>> get_goods_gallery_list_Map(Element goodsGallery) ;
//样本
public Map<String,String> get_attribute_swatches(Element swatches);
public List<String> get_attribute_swatches_list(Element swatches);
//尺寸
public Map<String,String> get_attribute_sizeContainer(Element sizeContainer);
public List<String> get_attribute_sizeContainer_list(Element sizeContainer);
//获取下一页列表
public String get_next_link(String href);
//遍历显示
public void MapForeach(Map<String,String> map);
public List<String> get_goods_gallery_list(Element goodsGallery);
//获取分类,用逗号分开
public String get_Category(Element category);
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/Article.java
package com.ehais.figoarticle.model;
import java.io.Serializable;
public class Article implements Serializable {
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.id
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Long id;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.site_id
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Long siteId;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.wid
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Long wid;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.gid
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Long gid;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.title
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private String title;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.author
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private String author;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.reply
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Integer reply;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.pre_reply
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Integer preReply;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.seply_status
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Integer seplyStatus;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.brand_id
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Integer brandId;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.positive
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Integer positive;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.status
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Integer status;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.url
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private String url;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.share_url
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private String shareUrl;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.release_time
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Integer releaseTime;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.created
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Integer created;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.updated
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Integer updated;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.deleted
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Integer deleted;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.keyword
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private String keyword;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.is_danger
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Integer isDanger;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.item_id
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private Long itemId;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column article.abstracts
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private String abstracts;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
private static final long serialVersionUID = 1L;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.id
*
* @return the value of article.id
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Long getId() {
return id;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.id
*
* @param id the value for article.id
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setId(Long id) {
this.id = id;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.site_id
*
* @return the value of article.site_id
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Long getSiteId() {
return siteId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.site_id
*
* @param siteId the value for article.site_id
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setSiteId(Long siteId) {
this.siteId = siteId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.wid
*
* @return the value of article.wid
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Long getWid() {
return wid;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.wid
*
* @param wid the value for article.wid
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setWid(Long wid) {
this.wid = wid;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.gid
*
* @return the value of article.gid
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Long getGid() {
return gid;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.gid
*
* @param gid the value for article.gid
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setGid(Long gid) {
this.gid = gid;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.title
*
* @return the value of article.title
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public String getTitle() {
return title;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.title
*
* @param title the value for article.title
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setTitle(String title) {
this.title = title;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.author
*
* @return the value of article.author
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public String getAuthor() {
return author;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.author
*
* @param author the value for article.author
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setAuthor(String author) {
this.author = author;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.reply
*
* @return the value of article.reply
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Integer getReply() {
return reply;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.reply
*
* @param reply the value for article.reply
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setReply(Integer reply) {
this.reply = reply;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.pre_reply
*
* @return the value of article.pre_reply
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Integer getPreReply() {
return preReply;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.pre_reply
*
* @param preReply the value for article.pre_reply
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setPreReply(Integer preReply) {
this.preReply = preReply;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.seply_status
*
* @return the value of article.seply_status
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Integer getSeplyStatus() {
return seplyStatus;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.seply_status
*
* @param seplyStatus the value for article.seply_status
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setSeplyStatus(Integer seplyStatus) {
this.seplyStatus = seplyStatus;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.brand_id
*
* @return the value of article.brand_id
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Integer getBrandId() {
return brandId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.brand_id
*
* @param brandId the value for article.brand_id
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setBrandId(Integer brandId) {
this.brandId = brandId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.positive
*
* @return the value of article.positive
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Integer getPositive() {
return positive;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.positive
*
* @param positive the value for article.positive
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setPositive(Integer positive) {
this.positive = positive;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.status
*
* @return the value of article.status
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Integer getStatus() {
return status;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.status
*
* @param status the value for article.status
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setStatus(Integer status) {
this.status = status;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.url
*
* @return the value of article.url
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public String getUrl() {
return url;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.url
*
* @param url the value for article.url
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setUrl(String url) {
this.url = url;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.share_url
*
* @return the value of article.share_url
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public String getShareUrl() {
return shareUrl;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.share_url
*
* @param shareUrl the value for article.share_url
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setShareUrl(String shareUrl) {
this.shareUrl = shareUrl;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.release_time
*
* @return the value of article.release_time
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Integer getReleaseTime() {
return releaseTime;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.release_time
*
* @param releaseTime the value for article.release_time
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setReleaseTime(Integer releaseTime) {
this.releaseTime = releaseTime;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.created
*
* @return the value of article.created
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Integer getCreated() {
return created;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.created
*
* @param created the value for article.created
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setCreated(Integer created) {
this.created = created;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.updated
*
* @return the value of article.updated
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Integer getUpdated() {
return updated;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.updated
*
* @param updated the value for article.updated
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setUpdated(Integer updated) {
this.updated = updated;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.deleted
*
* @return the value of article.deleted
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Integer getDeleted() {
return deleted;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.deleted
*
* @param deleted the value for article.deleted
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setDeleted(Integer deleted) {
this.deleted = deleted;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.keyword
*
* @return the value of article.keyword
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public String getKeyword() {
return keyword;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.keyword
*
* @param keyword the value for article.keyword
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setKeyword(String keyword) {
this.keyword = keyword;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.is_danger
*
* @return the value of article.is_danger
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Integer getIsDanger() {
return isDanger;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.is_danger
*
* @param isDanger the value for article.is_danger
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setIsDanger(Integer isDanger) {
this.isDanger = isDanger;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.item_id
*
* @return the value of article.item_id
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Long getItemId() {
return itemId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.item_id
*
* @param itemId the value for article.item_id
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setItemId(Long itemId) {
this.itemId = itemId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column article.abstracts
*
* @return the value of article.abstracts
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public String getAbstracts() {
return abstracts;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column article.abstracts
*
* @param abstracts the value for article.abstracts
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setAbstracts(String abstracts) {
this.abstracts = abstracts;
}
}<file_sep>/FigoShop/FigoShop.sql
<<<<<<< HEAD
/*
Navicat MySQL Data Transfer
Source Server : 车巧飞--头条
Source Server Version : 50173
Source Host : 172.16.58.3:3306
Source Database : yc
Target Server Type : MYSQL
Target Server Version : 50173
File Encoding : 65001
Date: 2017-05-17 19:36:53
*/
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- Table structure for `admin`
-- ----------------------------
DROP TABLE IF EXISTS `admin`;
CREATE TABLE `admin` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(100) NOT NULL,
`password` varchar(100) NOT NULL,
`nickname` varchar(255) DEFAULT NULL,
`phone` varchar(255) NOT NULL,
`created` int(11) DEFAULT '0',
`updated` int(11) DEFAULT '0',
`deleted` int(11) DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=4 DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of admin
-- ----------------------------
INSERT INTO `admin` VALUES ('1', 'admin', '<PASSWORD>', 'admin', '', '1439177754', '1439177754', '0');
INSERT INTO `admin` VALUES ('2', 'cky', 'e10adc3949ba59abbe56e057f20f883e', null, '13422323324', '1490842306', '0', '0');
INSERT INTO `admin` VALUES ('3', 'OPPO', '10fb6faf0bff4e694eaabb2d692b8d97', null, '', '1491389362', '0', '1');
-- ----------------------------
-- Table structure for `article`
-- ----------------------------
DROP TABLE IF EXISTS `article`;
CREATE TABLE `article` (
`id` bigint(20) NOT NULL AUTO_INCREMENT,
`site_id` bigint(20) NOT NULL,
`wid` bigint(20) NOT NULL,
`gid` bigint(20) NOT NULL,
`title` varchar(255) NOT NULL COMMENT '文章标题',
`author` varchar(255) NOT NULL COMMENT '作者',
`abstracts` text NOT NULL COMMENT '摘要',
`reply` int(11) NOT NULL DEFAULT '0' COMMENT '回复数',
`pre_reply` int(11) NOT NULL DEFAULT '0' COMMENT '上一次回复数',
`seply_status` int(11) NOT NULL DEFAULT '0' COMMENT '回复数状态 0为否 1为是',
`brand_id` int(11) NOT NULL DEFAULT '0' COMMENT '品牌ID,与brand表ID对应,0表示未定义',
`positive` int(11) NOT NULL DEFAULT '2' COMMENT '正负面 0为负 1为正 2未标识',
`status` int(11) NOT NULL DEFAULT '0' COMMENT '文章状态 0 未处理 1处理中 2 处理完成',
`url` varchar(500) NOT NULL COMMENT '原文URL',
`share_url` varchar(500) NOT NULL COMMENT '分享URL',
`release_time` int(11) NOT NULL COMMENT '发布时间',
`created` int(11) NOT NULL DEFAULT '0',
`updated` int(11) NOT NULL DEFAULT '0',
`deleted` int(11) NOT NULL DEFAULT '0',
`keyword` varchar(200) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `site_id` (`site_id`),
CONSTRAINT `article_ibfk_1` FOREIGN KEY (`site_id`) REFERENCES `site_desc` (`id`) ON UPDATE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COMMENT='文章列表';
-- ----------------------------
-- Records of article
-- ----------------------------
-- ----------------------------
-- Table structure for `article_comment`
-- ----------------------------
DROP TABLE IF EXISTS `article_comment`;
CREATE TABLE `article_comment` (
`order_id` int(11) NOT NULL AUTO_INCREMENT,
`article_id` bigint(20) NOT NULL,
`comment_content` varchar(255) NOT NULL,
`status` int(11) NOT NULL DEFAULT '1',
`deleted` int(11) NOT NULL DEFAULT '0',
`created` int(11) NOT NULL DEFAULT '0',
`updated` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`order_id`)
) ENGINE=InnoDB AUTO_INCREMENT=8 DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of article_comment
-- ----------------------------
-- ----------------------------
-- Table structure for `brand`
-- ----------------------------
DROP TABLE IF EXISTS `brand`;
CREATE TABLE `brand` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) NOT NULL COMMENT '品牌名称',
`created` int(11) NOT NULL DEFAULT '0',
`updated` int(11) NOT NULL DEFAULT '0',
`deleted` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=9 DEFAULT CHARSET=utf8 COMMENT='品牌表';
-- ----------------------------
-- Records of brand
-- ----------------------------
INSERT INTO `brand` VALUES ('1', '小米', '1489398175', '0', '0');
INSERT INTO `brand` VALUES ('3', '魅族', '1489563441', '1489563451', '0');
INSERT INTO `brand` VALUES ('4', '华为', '1489758141', '0', '0');
INSERT INTO `brand` VALUES ('5', 'Apple', '1489772260', '0', '0');
INSERT INTO `brand` VALUES ('6', 'vivo', '1489772364', '1489916670', '0');
INSERT INTO `brand` VALUES ('7', 'OPPO', '1489926404', '0', '0');
INSERT INTO `brand` VALUES ('8', '三星', '1490162255', '0', '0');
-- ----------------------------
-- Table structure for `collect`
-- ----------------------------
DROP TABLE IF EXISTS `collect`;
CREATE TABLE `collect` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`task` varchar(255) NOT NULL,
`title` varchar(255) DEFAULT NULL,
`url` varchar(255) NOT NULL COMMENT '链接',
`release_time` int(11) NOT NULL DEFAULT '0' COMMENT '发布时间',
`type` int(11) NOT NULL DEFAULT '1' COMMENT '来源 1=网页 2=新闻',
`search_time` int(11) NOT NULL DEFAULT '0' COMMENT '搜索时间',
`platform` varchar(255) NOT NULL,
`brand` varchar(255) NOT NULL,
`record_num` int(11) NOT NULL DEFAULT '0' COMMENT '返回记录数',
`status` int(1) NOT NULL DEFAULT '0',
`created` int(11) NOT NULL DEFAULT '0',
`updated` int(11) NOT NULL DEFAULT '0',
`deleted` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=16928 DEFAULT CHARSET=utf8 COMMENT='搜索记录表';
-- ----------------------------
-- Records of collect
-- ----------------------------
-- ----------------------------
-- Table structure for `event_log`
-- ----------------------------
DROP TABLE IF EXISTS `event_log`;
CREATE TABLE `event_log` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) NOT NULL,
`event` varchar(255) NOT NULL,
`ip` varchar(255) NOT NULL,
`created` int(11) NOT NULL DEFAULT '0',
`updated` int(11) NOT NULL DEFAULT '0',
`deleted` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=7689 DEFAULT CHARSET=utf8 COMMENT='日志表';
-- ----------------------------
-- Records of event_log
-- ----------------------------
-- ----------------------------
-- Table structure for `excel`
-- ----------------------------
DROP TABLE IF EXISTS `excel`;
CREATE TABLE `excel` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`task` varchar(255) NOT NULL,
`title` varchar(255) DEFAULT NULL,
`url` varchar(255) NOT NULL COMMENT '链接',
`release_time` int(11) NOT NULL DEFAULT '0' COMMENT '发布时间',
`search_time` int(11) NOT NULL DEFAULT '0' COMMENT '最新搜索时间',
`platform` varchar(255) NOT NULL,
`brand` varchar(255) NOT NULL,
`created` int(11) NOT NULL DEFAULT '0',
`updated` int(11) NOT NULL DEFAULT '0',
`deleted` int(11) NOT NULL DEFAULT '0',
`valid` int(11) DEFAULT '1',
`baidu_count` int(11) DEFAULT NULL,
`news_count` int(11) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=7870 DEFAULT CHARSET=utf8 COMMENT='导入链接表';
-- ----------------------------
-- Records of excel
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_brand`
-- ----------------------------
DROP TABLE IF EXISTS `hai_brand`;
CREATE TABLE `hai_brand` (
`brand_id` int(11) unsigned NOT NULL AUTO_INCREMENT COMMENT '自动编号',
`brand_name` varchar(60) NOT NULL DEFAULT '' COMMENT '品牌名称',
`brand_logo` varchar(500) NOT NULL DEFAULT '' COMMENT '品牌logo',
`brand_desc` text NOT NULL COMMENT '品牌描述',
`site_url` varchar(255) NOT NULL DEFAULT '' COMMENT '连接地址',
`sort_order` smallint(3) unsigned NOT NULL DEFAULT '50' COMMENT '排序',
`is_show` tinyint(1) unsigned NOT NULL DEFAULT '1' COMMENT '是否显示',
PRIMARY KEY (`brand_id`),
KEY `is_show` (`is_show`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_brand
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_category`
-- ----------------------------
DROP TABLE IF EXISTS `hai_category`;
CREATE TABLE `hai_category` (
`cat_id` int(11) unsigned NOT NULL AUTO_INCREMENT COMMENT '分类编号',
`cat_name` varchar(90) NOT NULL DEFAULT '' COMMENT '分类名称',
`keywords` varchar(255) NOT NULL DEFAULT '' COMMENT '关键字',
`cat_desc` varchar(255) NOT NULL DEFAULT '' COMMENT '分类简介',
`parent_id` int(11) unsigned NOT NULL DEFAULT '0' COMMENT '所属上级分类',
`sort_order` smallint(1) unsigned NOT NULL DEFAULT '0' COMMENT '排序',
`is_show` tinyint(1) unsigned NOT NULL DEFAULT '1' COMMENT '是否显示',
`brand_id` text COMMENT '品牌编号',
`category_url` varchar(200) DEFAULT NULL COMMENT '分类链接地址',
PRIMARY KEY (`cat_id`),
KEY `parent_id` (`parent_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_category
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods`;
CREATE TABLE `hai_goods` (
`goods_id` bigint(8) unsigned NOT NULL AUTO_INCREMENT COMMENT '商品编号',
`cat_id` int(5) unsigned NOT NULL DEFAULT '0' COMMENT '分类编号',
`goods_sn` varchar(60) NOT NULL DEFAULT '' COMMENT '商品编码',
`goods_name` varchar(120) NOT NULL DEFAULT '' COMMENT '商品名称',
`brand_id` int(5) unsigned NOT NULL DEFAULT '0' COMMENT '品牌编号',
`shop_price` int(10) unsigned NOT NULL DEFAULT '0' COMMENT '商品价格',
`keywords` varchar(255) NOT NULL DEFAULT '' COMMENT '关键字',
`goods_brief` text NOT NULL COMMENT '商品简介',
`goods_desc` text NOT NULL COMMENT '商品详情',
`act_desc` varchar(100) DEFAULT NULL COMMENT '标题旁边的标注',
`goods_thumb` varchar(255) NOT NULL DEFAULT '' COMMENT '缩略图',
`goods_img` varchar(255) NOT NULL DEFAULT '' COMMENT '商品图片',
`original_img` varchar(255) NOT NULL DEFAULT '' COMMENT '商品原图',
`is_on_sale` tinyint(1) unsigned NOT NULL DEFAULT '1' COMMENT '是否出售中',
`add_time` int(10) unsigned NOT NULL DEFAULT '0' COMMENT '添加时间',
`sort_order` smallint(4) unsigned NOT NULL DEFAULT '100' COMMENT '排序',
`last_update` int(10) unsigned NOT NULL DEFAULT '0' COMMENT '最后更新时间',
`exchangeRate` decimal(10,4) DEFAULT '0.0000' COMMENT '兑换率',
`profit` decimal(10,4) DEFAULT '0.0000' COMMENT '利益',
`shipping` decimal(10,4) DEFAULT '0.0000' COMMENT '运费',
`profitType` int(2) DEFAULT '0' COMMENT '0:乘以折扣,1:加上费用',
`currency` varchar(20) DEFAULT NULL COMMENT '货币类型',
`goods_url` varchar(500) DEFAULT NULL COMMENT '网上抓取的网址',
PRIMARY KEY (`goods_id`),
KEY `goods_sn` (`goods_sn`),
KEY `cat_id` (`cat_id`),
KEY `last_update` (`last_update`),
KEY `brand_id` (`brand_id`),
KEY `sort_order` (`sort_order`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods_attr`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods_attr`;
CREATE TABLE `hai_goods_attr` (
`goods_attr_id` int(10) unsigned NOT NULL AUTO_INCREMENT COMMENT '属性编号',
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0' COMMENT '商品编号',
`attr_value` text NOT NULL COMMENT '属性名称',
`attr_price` varchar(255) NOT NULL DEFAULT '' COMMENT '属性价格',
PRIMARY KEY (`goods_attr_id`),
KEY `goods_id` (`goods_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods_attr
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods_gallery`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods_gallery`;
CREATE TABLE `hai_goods_gallery` (
`img_id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`goods_id` bigint(20) unsigned NOT NULL DEFAULT '0' COMMENT '商品编号',
`img_url` varchar(255) NOT NULL DEFAULT '' COMMENT '在图地址',
`img_desc` varchar(255) NOT NULL DEFAULT '' COMMENT '图片描述',
`thumb_url` varchar(255) NOT NULL DEFAULT '' COMMENT '缩略图地址',
`img_original` varchar(255) NOT NULL DEFAULT '' COMMENT '原图地址',
PRIMARY KEY (`img_id`),
KEY `goods_id` (`goods_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods_gallery
-- ----------------------------
CREATE TABLE `hai_goods_url` (
`goods_url_id` int(11) NOT NULL AUTO_INCREMENT ,
`cat_id` int(11) NULL DEFAULT NULL ,
`goods_url` varchar(500) CHARACTER SET utf8 COLLATE utf8_general_ci NULL DEFAULT NULL ,
PRIMARY KEY (`goods_url_id`)
)
ENGINE=InnoDB
DEFAULT CHARACTER SET=utf8 COLLATE=utf8_general_ci
AUTO_INCREMENT=1
ROW_FORMAT=COMPACT
;
=======
/*
Navicat MySQL Data Transfer
Source Server : 车巧飞--头条
Source Server Version : 50173
Source Host : 120.27.214.29:3306
Source Database : yc
Target Server Type : MYSQL
Target Server Version : 50173
File Encoding : 65001
Date: 2017-05-17 19:36:53
*/
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- Table structure for `admin`
-- ----------------------------
DROP TABLE IF EXISTS `admin`;
CREATE TABLE `admin` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(100) NOT NULL,
`password` varchar(100) NOT NULL,
`nickname` varchar(255) DEFAULT NULL,
`phone` varchar(255) NOT NULL,
`created` int(11) DEFAULT '0',
`updated` int(11) DEFAULT '0',
`deleted` int(11) DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=4 DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of admin
-- ----------------------------
INSERT INTO `admin` VALUES ('1', 'admin', '8d423d5d06259f85af89054728f216c9', 'admin', '', '1439177754', '1439177754', '0');
INSERT INTO `admin` VALUES ('2', 'cky', 'e10adc3949ba59abbe56e057f20f883e', null, '13422323324', '1490842306', '0', '0');
INSERT INTO `admin` VALUES ('3', 'OPPO', '<PASSWORD>', null, '', '1491389362', '0', '1');
-- ----------------------------
-- Table structure for `article`
-- ----------------------------
DROP TABLE IF EXISTS `article`;
CREATE TABLE `article` (
`id` bigint(20) NOT NULL AUTO_INCREMENT,
`site_id` bigint(20) NOT NULL,
`wid` bigint(20) NOT NULL,
`gid` bigint(20) NOT NULL,
`title` varchar(255) NOT NULL COMMENT '文章标题',
`author` varchar(255) NOT NULL COMMENT '作者',
`abstracts` text NOT NULL COMMENT '摘要',
`reply` int(11) NOT NULL DEFAULT '0' COMMENT '回复数',
`pre_reply` int(11) NOT NULL DEFAULT '0' COMMENT '上一次回复数',
`seply_status` int(11) NOT NULL DEFAULT '0' COMMENT '回复数状态 0为否 1为是',
`brand_id` int(11) NOT NULL DEFAULT '0' COMMENT '品牌ID,与brand表ID对应,0表示未定义',
`positive` int(11) NOT NULL DEFAULT '2' COMMENT '正负面 0为负 1为正 2未标识',
`status` int(11) NOT NULL DEFAULT '0' COMMENT '文章状态 0 未处理 1处理中 2 处理完成',
`url` varchar(500) NOT NULL COMMENT '原文URL',
`share_url` varchar(500) NOT NULL COMMENT '分享URL',
`release_time` int(11) NOT NULL COMMENT '发布时间',
`created` int(11) NOT NULL DEFAULT '0',
`updated` int(11) NOT NULL DEFAULT '0',
`deleted` int(11) NOT NULL DEFAULT '0',
`keyword` varchar(200) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `site_id` (`site_id`),
CONSTRAINT `article_ibfk_1` FOREIGN KEY (`site_id`) REFERENCES `site_desc` (`id`) ON UPDATE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COMMENT='文章列表';
-- ----------------------------
-- Records of article
-- ----------------------------
-- ----------------------------
-- Table structure for `article_comment`
-- ----------------------------
DROP TABLE IF EXISTS `article_comment`;
CREATE TABLE `article_comment` (
`order_id` int(11) NOT NULL AUTO_INCREMENT,
`article_id` bigint(20) NOT NULL,
`comment_content` varchar(255) NOT NULL,
`status` int(11) NOT NULL DEFAULT '1',
`deleted` int(11) NOT NULL DEFAULT '0',
`created` int(11) NOT NULL DEFAULT '0',
`updated` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`order_id`)
) ENGINE=InnoDB AUTO_INCREMENT=8 DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of article_comment
-- ----------------------------
-- ----------------------------
-- Table structure for `brand`
-- ----------------------------
DROP TABLE IF EXISTS `brand`;
CREATE TABLE `brand` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) NOT NULL COMMENT '品牌名称',
`created` int(11) NOT NULL DEFAULT '0',
`updated` int(11) NOT NULL DEFAULT '0',
`deleted` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=9 DEFAULT CHARSET=utf8 COMMENT='品牌表';
-- ----------------------------
-- Records of brand
-- ----------------------------
INSERT INTO `brand` VALUES ('1', '小米', '1489398175', '0', '0');
INSERT INTO `brand` VALUES ('3', '魅族', '1489563441', '1489563451', '0');
INSERT INTO `brand` VALUES ('4', '华为', '1489758141', '0', '0');
INSERT INTO `brand` VALUES ('5', 'Apple', '1489772260', '0', '0');
INSERT INTO `brand` VALUES ('6', 'vivo', '1489772364', '1489916670', '0');
INSERT INTO `brand` VALUES ('7', 'OPPO', '1489926404', '0', '0');
INSERT INTO `brand` VALUES ('8', '三星', '1490162255', '0', '0');
-- ----------------------------
-- Table structure for `collect`
-- ----------------------------
DROP TABLE IF EXISTS `collect`;
CREATE TABLE `collect` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`task` varchar(255) NOT NULL,
`title` varchar(255) DEFAULT NULL,
`url` varchar(255) NOT NULL COMMENT '链接',
`release_time` int(11) NOT NULL DEFAULT '0' COMMENT '发布时间',
`type` int(11) NOT NULL DEFAULT '1' COMMENT '来源 1=网页 2=新闻',
`search_time` int(11) NOT NULL DEFAULT '0' COMMENT '搜索时间',
`platform` varchar(255) NOT NULL,
`brand` varchar(255) NOT NULL,
`record_num` int(11) NOT NULL DEFAULT '0' COMMENT '返回记录数',
`status` int(1) NOT NULL DEFAULT '0',
`created` int(11) NOT NULL DEFAULT '0',
`updated` int(11) NOT NULL DEFAULT '0',
`deleted` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=16928 DEFAULT CHARSET=utf8 COMMENT='搜索记录表';
-- ----------------------------
-- Records of collect
-- ----------------------------
-- ----------------------------
-- Table structure for `event_log`
-- ----------------------------
DROP TABLE IF EXISTS `event_log`;
CREATE TABLE `event_log` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) NOT NULL,
`event` varchar(255) NOT NULL,
`ip` varchar(255) NOT NULL,
`created` int(11) NOT NULL DEFAULT '0',
`updated` int(11) NOT NULL DEFAULT '0',
`deleted` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=7689 DEFAULT CHARSET=utf8 COMMENT='日志表';
-- ----------------------------
-- Records of event_log
-- ----------------------------
-- ----------------------------
-- Table structure for `excel`
-- ----------------------------
DROP TABLE IF EXISTS `excel`;
CREATE TABLE `excel` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`task` varchar(255) NOT NULL,
`title` varchar(255) DEFAULT NULL,
`url` varchar(255) NOT NULL COMMENT '链接',
`release_time` int(11) NOT NULL DEFAULT '0' COMMENT '发布时间',
`search_time` int(11) NOT NULL DEFAULT '0' COMMENT '最新搜索时间',
`platform` varchar(255) NOT NULL,
`brand` varchar(255) NOT NULL,
`created` int(11) NOT NULL DEFAULT '0',
`updated` int(11) NOT NULL DEFAULT '0',
`deleted` int(11) NOT NULL DEFAULT '0',
`valid` int(11) DEFAULT '1',
`baidu_count` int(11) DEFAULT NULL,
`news_count` int(11) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=7870 DEFAULT CHARSET=utf8 COMMENT='导入链接表';
-- ----------------------------
-- Records of excel
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_brand`
-- ----------------------------
DROP TABLE IF EXISTS `hai_brand`;
CREATE TABLE `hai_brand` (
`brand_id` int(11) unsigned NOT NULL AUTO_INCREMENT COMMENT '自动编号',
`brand_name` varchar(60) NOT NULL DEFAULT '' COMMENT '品牌名称',
`brand_logo` varchar(500) NOT NULL DEFAULT '' COMMENT '品牌logo',
`brand_desc` text NOT NULL COMMENT '品牌描述',
`site_url` varchar(255) NOT NULL DEFAULT '' COMMENT '连接地址',
`sort_order` smallint(3) unsigned NOT NULL DEFAULT '50' COMMENT '排序',
`is_show` tinyint(1) unsigned NOT NULL DEFAULT '1' COMMENT '是否显示',
PRIMARY KEY (`brand_id`),
KEY `is_show` (`is_show`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_brand
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_category`
-- ----------------------------
DROP TABLE IF EXISTS `hai_category`;
CREATE TABLE `hai_category` (
`cat_id` int(11) unsigned NOT NULL AUTO_INCREMENT COMMENT '分类编号',
`cat_name` varchar(90) NOT NULL DEFAULT '' COMMENT '分类名称',
`keywords` varchar(255) NOT NULL DEFAULT '' COMMENT '关键字',
`cat_desc` varchar(255) NOT NULL DEFAULT '' COMMENT '分类简介',
`parent_id` int(11) unsigned NOT NULL DEFAULT '0' COMMENT '所属上级分类',
`sort_order` smallint(1) unsigned NOT NULL DEFAULT '0' COMMENT '排序',
`is_show` tinyint(1) unsigned NOT NULL DEFAULT '1' COMMENT '是否显示',
`brand_id` text COMMENT '品牌编号',
`category_url` varchar(200) DEFAULT NULL COMMENT '分类链接地址',
PRIMARY KEY (`cat_id`),
KEY `parent_id` (`parent_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_category
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods`;
CREATE TABLE `hai_goods` (
`goods_id` bigint(8) unsigned NOT NULL AUTO_INCREMENT COMMENT '商品编号',
`cat_id` int(5) unsigned NOT NULL DEFAULT '0' COMMENT '分类编号',
`goods_sn` varchar(60) NOT NULL DEFAULT '' COMMENT '商品编码',
`goods_name` varchar(120) NOT NULL DEFAULT '' COMMENT '商品名称',
`brand_id` int(5) unsigned NOT NULL DEFAULT '0' COMMENT '品牌编号',
`shop_price` int(10) unsigned NOT NULL DEFAULT '0' COMMENT '商品价格',
`keywords` varchar(255) NOT NULL DEFAULT '' COMMENT '关键字',
`goods_brief` text NOT NULL COMMENT '商品简介',
`goods_desc` text NOT NULL COMMENT '商品详情',
`act_desc` varchar(100) DEFAULT NULL COMMENT '标题旁边的标注',
`goods_thumb` varchar(255) NOT NULL DEFAULT '' COMMENT '缩略图',
`goods_img` varchar(255) NOT NULL DEFAULT '' COMMENT '商品图片',
`original_img` varchar(255) NOT NULL DEFAULT '' COMMENT '商品原图',
`is_on_sale` tinyint(1) unsigned NOT NULL DEFAULT '1' COMMENT '是否出售中',
`add_time` int(10) unsigned NOT NULL DEFAULT '0' COMMENT '添加时间',
`sort_order` smallint(4) unsigned NOT NULL DEFAULT '100' COMMENT '排序',
`last_update` int(10) unsigned NOT NULL DEFAULT '0' COMMENT '最后更新时间',
`exchangeRate` decimal(10,4) DEFAULT '0.0000' COMMENT '兑换率',
`profit` decimal(10,4) DEFAULT '0.0000' COMMENT '利益',
`shipping` decimal(10,4) DEFAULT '0.0000' COMMENT '运费',
`profitType` int(2) DEFAULT '0' COMMENT '0:乘以折扣,1:加上费用',
`currency` varchar(20) DEFAULT NULL COMMENT '货币类型',
`goods_url` varchar(500) DEFAULT NULL COMMENT '网上抓取的网址',
PRIMARY KEY (`goods_id`),
KEY `goods_sn` (`goods_sn`),
KEY `cat_id` (`cat_id`),
KEY `last_update` (`last_update`),
KEY `brand_id` (`brand_id`),
KEY `sort_order` (`sort_order`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods_attr`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods_attr`;
CREATE TABLE `hai_goods_attr` (
`goods_attr_id` int(10) unsigned NOT NULL AUTO_INCREMENT COMMENT '属性编号',
`goods_id` mediumint(8) unsigned NOT NULL DEFAULT '0' COMMENT '商品编号',
`attr_value` text NOT NULL COMMENT '属性名称',
`attr_price` varchar(255) NOT NULL DEFAULT '' COMMENT '属性价格',
`attr_type` varchar(255) NOT NULL DEFAULT '' COMMENT '属性类型',
PRIMARY KEY (`goods_attr_id`),
KEY `goods_id` (`goods_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods_attr
-- ----------------------------
-- ----------------------------
-- Table structure for `hai_goods_gallery`
-- ----------------------------
DROP TABLE IF EXISTS `hai_goods_gallery`;
CREATE TABLE `hai_goods_gallery` (
`img_id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`goods_id` bigint(20) unsigned NOT NULL DEFAULT '0' COMMENT '商品编号',
`img_url` varchar(255) NOT NULL DEFAULT '' COMMENT '在图地址',
`img_desc` varchar(255) NOT NULL DEFAULT '' COMMENT '图片描述',
`thumb_url` varchar(255) NOT NULL DEFAULT '' COMMENT '缩略图地址',
`img_original` varchar(255) NOT NULL DEFAULT '' COMMENT '原图地址',
PRIMARY KEY (`img_id`),
KEY `goods_id` (`goods_id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- ----------------------------
-- Records of hai_goods_gallery
-- ----------------------------
>>>>>>> 75b31b492b4d4ba9673e4c2ab624ddf56b2bb437
<file_sep>/school-weixin/sql/activity.sql
create table activityList
(
activityName varchar(100) not null,
pCount int not null,
startTime timestamp not null,
endTime timestamp not null,
finalTime timestamp not null,
id int primary not null
);
create table activityDetail
(
id int primary,
name varchar(40),
foreign key(id) references activityList(id)
);<file_sep>/eh-weixin/src/main/java/org/ehais/weixin/model/WxNotityPay.java
package org.ehais.weixin.model;
public class WxNotityPay {
public WxNotityPay() {
super();
// TODO Auto-generated constructor stub
}
public WxNotityPay(WeiXinNotifyPay model) {
super();
this.returnCode = model.getReturn_code();
this.returnMsg = model.getReturn_msg();
this.appid = model.getAppid();
this.mchId = model.getMch_id();
this.deviceInfo = model.getDevice_info();
this.nonceStr = model.getNonce_str();
this.sign = model.getSign();
this.resultCode = model.getResult_code();
this.errCode = model.getErr_code();
this.errCodeDes = model.getErr_code_des();
this.openid = model.getOpenid();
this.isSubscribe = model.getIs_subscribe();
this.tradeType = model.getTrade_type();
this.bankType = model.getBank_type();
this.totalFee = model.getTotal_fee();
this.feeType = model.getFee_type();
this.cashFee = model.getCash_fee();
this.cashFeeType = model.getCash_fee_type();
this.couponFee = model.getCoupon_fee();
this.couponCount = model.getCoupon_count();
this.couponId1 = model.getCoupon_id_1();
this.couponFee1 = model.getCoupon_fee_1();
this.transactionId = model.getTransaction_id();
this.outTradeNo = model.getOut_trade_no();
this.attach = model.getAttach();
this.timeEnd = model.getTime_end();
}
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.notify_pay_id
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private Integer notifyPayId;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.return_code
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String returnCode;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.return_msg
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String returnMsg;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.appid
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String appid;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.mch_id
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String mchId;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.device_info
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String deviceInfo;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.nonce_str
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String nonceStr;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.sign
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String sign;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.result_code
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String resultCode;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.err_code
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String errCode;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.err_code_des
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String errCodeDes;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.openid
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String openid;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.is_subscribe
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String isSubscribe;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.trade_type
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String tradeType;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.bank_type
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String bankType;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.total_fee
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private Integer totalFee;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.fee_type
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String feeType;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.cash_fee
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private Integer cashFee;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.cash_fee_type
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String cashFeeType;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.coupon_fee
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private Integer couponFee;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.coupon_count
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private Integer couponCount;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.coupon_id_1
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String couponId1;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.coupon_fee_1
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private Integer couponFee1;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.transaction_id
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String transactionId;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.out_trade_no
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String outTradeNo;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.attach
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private String attach;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_notity_pay.time_end
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
private Float timeEnd;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.notify_pay_id
*
* @return the value of wx_notity_pay.notify_pay_id
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public Integer getNotifyPayId() {
return notifyPayId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.notify_pay_id
*
* @param notifyPayId the value for wx_notity_pay.notify_pay_id
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setNotifyPayId(Integer notifyPayId) {
this.notifyPayId = notifyPayId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.return_code
*
* @return the value of wx_notity_pay.return_code
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getReturnCode() {
return returnCode;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.return_code
*
* @param returnCode the value for wx_notity_pay.return_code
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setReturnCode(String returnCode) {
this.returnCode = returnCode;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.return_msg
*
* @return the value of wx_notity_pay.return_msg
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getReturnMsg() {
return returnMsg;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.return_msg
*
* @param returnMsg the value for wx_notity_pay.return_msg
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setReturnMsg(String returnMsg) {
this.returnMsg = returnMsg;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.appid
*
* @return the value of wx_notity_pay.appid
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getAppid() {
return appid;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.appid
*
* @param appid the value for wx_notity_pay.appid
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setAppid(String appid) {
this.appid = appid;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.mch_id
*
* @return the value of wx_notity_pay.mch_id
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getMchId() {
return mchId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.mch_id
*
* @param mchId the value for wx_notity_pay.mch_id
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setMchId(String mchId) {
this.mchId = mchId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.device_info
*
* @return the value of wx_notity_pay.device_info
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getDeviceInfo() {
return deviceInfo;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.device_info
*
* @param deviceInfo the value for wx_notity_pay.device_info
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setDeviceInfo(String deviceInfo) {
this.deviceInfo = deviceInfo;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.nonce_str
*
* @return the value of wx_notity_pay.nonce_str
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getNonceStr() {
return nonceStr;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.nonce_str
*
* @param nonceStr the value for wx_notity_pay.nonce_str
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setNonceStr(String nonceStr) {
this.nonceStr = nonceStr;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.sign
*
* @return the value of wx_notity_pay.sign
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getSign() {
return sign;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.sign
*
* @param sign the value for wx_notity_pay.sign
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setSign(String sign) {
this.sign = sign;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.result_code
*
* @return the value of wx_notity_pay.result_code
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getResultCode() {
return resultCode;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.result_code
*
* @param resultCode the value for wx_notity_pay.result_code
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setResultCode(String resultCode) {
this.resultCode = resultCode;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.err_code
*
* @return the value of wx_notity_pay.err_code
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getErrCode() {
return errCode;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.err_code
*
* @param errCode the value for wx_notity_pay.err_code
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setErrCode(String errCode) {
this.errCode = errCode;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.err_code_des
*
* @return the value of wx_notity_pay.err_code_des
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getErrCodeDes() {
return errCodeDes;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.err_code_des
*
* @param errCodeDes the value for wx_notity_pay.err_code_des
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setErrCodeDes(String errCodeDes) {
this.errCodeDes = errCodeDes;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.openid
*
* @return the value of wx_notity_pay.openid
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getOpenid() {
return openid;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.openid
*
* @param openid the value for wx_notity_pay.openid
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setOpenid(String openid) {
this.openid = openid;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.is_subscribe
*
* @return the value of wx_notity_pay.is_subscribe
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getIsSubscribe() {
return isSubscribe;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.is_subscribe
*
* @param isSubscribe the value for wx_notity_pay.is_subscribe
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setIsSubscribe(String isSubscribe) {
this.isSubscribe = isSubscribe;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.trade_type
*
* @return the value of wx_notity_pay.trade_type
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getTradeType() {
return tradeType;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.trade_type
*
* @param tradeType the value for wx_notity_pay.trade_type
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setTradeType(String tradeType) {
this.tradeType = tradeType;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.bank_type
*
* @return the value of wx_notity_pay.bank_type
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getBankType() {
return bankType;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.bank_type
*
* @param bankType the value for wx_notity_pay.bank_type
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setBankType(String bankType) {
this.bankType = bankType;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.total_fee
*
* @return the value of wx_notity_pay.total_fee
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public Integer getTotalFee() {
return totalFee;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.total_fee
*
* @param totalFee the value for wx_notity_pay.total_fee
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setTotalFee(Integer totalFee) {
this.totalFee = totalFee;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.fee_type
*
* @return the value of wx_notity_pay.fee_type
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getFeeType() {
return feeType;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.fee_type
*
* @param feeType the value for wx_notity_pay.fee_type
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setFeeType(String feeType) {
this.feeType = feeType;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.cash_fee
*
* @return the value of wx_notity_pay.cash_fee
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public Integer getCashFee() {
return cashFee;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.cash_fee
*
* @param cashFee the value for wx_notity_pay.cash_fee
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setCashFee(Integer cashFee) {
this.cashFee = cashFee;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.cash_fee_type
*
* @return the value of wx_notity_pay.cash_fee_type
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getCashFeeType() {
return cashFeeType;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.cash_fee_type
*
* @param cashFeeType the value for wx_notity_pay.cash_fee_type
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setCashFeeType(String cashFeeType) {
this.cashFeeType = cashFeeType;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.coupon_fee
*
* @return the value of wx_notity_pay.coupon_fee
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public Integer getCouponFee() {
return couponFee;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.coupon_fee
*
* @param couponFee the value for wx_notity_pay.coupon_fee
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setCouponFee(Integer couponFee) {
this.couponFee = couponFee;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.coupon_count
*
* @return the value of wx_notity_pay.coupon_count
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public Integer getCouponCount() {
return couponCount;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.coupon_count
*
* @param couponCount the value for wx_notity_pay.coupon_count
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setCouponCount(Integer couponCount) {
this.couponCount = couponCount;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.coupon_id_1
*
* @return the value of wx_notity_pay.coupon_id_1
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getCouponId1() {
return couponId1;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.coupon_id_1
*
* @param couponId1 the value for wx_notity_pay.coupon_id_1
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setCouponId1(String couponId1) {
this.couponId1 = couponId1;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.coupon_fee_1
*
* @return the value of wx_notity_pay.coupon_fee_1
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public Integer getCouponFee1() {
return couponFee1;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.coupon_fee_1
*
* @param couponFee1 the value for wx_notity_pay.coupon_fee_1
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setCouponFee1(Integer couponFee1) {
this.couponFee1 = couponFee1;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.transaction_id
*
* @return the value of wx_notity_pay.transaction_id
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getTransactionId() {
return transactionId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.transaction_id
*
* @param transactionId the value for wx_notity_pay.transaction_id
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setTransactionId(String transactionId) {
this.transactionId = transactionId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.out_trade_no
*
* @return the value of wx_notity_pay.out_trade_no
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getOutTradeNo() {
return outTradeNo;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.out_trade_no
*
* @param outTradeNo the value for wx_notity_pay.out_trade_no
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setOutTradeNo(String outTradeNo) {
this.outTradeNo = outTradeNo;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.attach
*
* @return the value of wx_notity_pay.attach
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public String getAttach() {
return attach;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.attach
*
* @param attach the value for wx_notity_pay.attach
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setAttach(String attach) {
this.attach = attach;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_notity_pay.time_end
*
* @return the value of wx_notity_pay.time_end
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public Float getTimeEnd() {
return timeEnd;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_notity_pay.time_end
*
* @param timeEnd the value for wx_notity_pay.time_end
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
public void setTimeEnd(Float timeEnd) {
this.timeEnd = timeEnd;
}
}<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/HaiWebsite.java
package com.ehais.figoarticle.model;
import java.io.Serializable;
public class HaiWebsite implements Serializable {
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_website.website_id
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
private Integer websiteId;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_website.website_name
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
private String websiteName;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_website.website_domain
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
private String websiteDomain;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_website.created
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
private Integer created;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_website.updated
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
private Integer updated;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_website.deleted
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
private Integer deleted;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_website
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
private static final long serialVersionUID = 1L;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_website.website_id
*
* @return the value of hai_website.website_id
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
public Integer getWebsiteId() {
return websiteId;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_website.website_id
*
* @param websiteId the value for hai_website.website_id
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
public void setWebsiteId(Integer websiteId) {
this.websiteId = websiteId;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_website.website_name
*
* @return the value of hai_website.website_name
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
public String getWebsiteName() {
return websiteName;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_website.website_name
*
* @param websiteName the value for hai_website.website_name
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
public void setWebsiteName(String websiteName) {
this.websiteName = websiteName;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_website.website_domain
*
* @return the value of hai_website.website_domain
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
public String getWebsiteDomain() {
return websiteDomain;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_website.website_domain
*
* @param websiteDomain the value for hai_website.website_domain
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
public void setWebsiteDomain(String websiteDomain) {
this.websiteDomain = websiteDomain;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_website.created
*
* @return the value of hai_website.created
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
public Integer getCreated() {
return created;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_website.created
*
* @param created the value for hai_website.created
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
public void setCreated(Integer created) {
this.created = created;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_website.updated
*
* @return the value of hai_website.updated
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
public Integer getUpdated() {
return updated;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_website.updated
*
* @param updated the value for hai_website.updated
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
public void setUpdated(Integer updated) {
this.updated = updated;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_website.deleted
*
* @return the value of hai_website.deleted
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
public Integer getDeleted() {
return deleted;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_website.deleted
*
* @param deleted the value for hai_website.deleted
*
* @mbg.generated Sat Jun 03 18:53:36 CST 2017
*/
public void setDeleted(Integer deleted) {
this.deleted = deleted;
}
}<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/HaiGoodsWithBLOBs.java
package com.ehais.figoarticle.model;
import java.io.Serializable;
public class HaiGoodsWithBLOBs extends HaiGoods implements Serializable {
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.goods_brief
*
* @mbg.generated Sun May 28 01:02:22 CST 2017
*/
private String goodsBrief;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column hai_goods.goods_desc
*
* @mbg.generated Sun May 28 01:02:22 CST 2017
*/
private String goodsDesc;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table hai_goods
*
* @mbg.generated Sun May 28 01:02:22 CST 2017
*/
private static final long serialVersionUID = 1L;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.goods_brief
*
* @return the value of hai_goods.goods_brief
*
* @mbg.generated Sun May 28 01:02:22 CST 2017
*/
public String getGoodsBrief() {
return goodsBrief;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.goods_brief
*
* @param goodsBrief the value for hai_goods.goods_brief
*
* @mbg.generated Sun May 28 01:02:22 CST 2017
*/
public void setGoodsBrief(String goodsBrief) {
this.goodsBrief = goodsBrief;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column hai_goods.goods_desc
*
* @return the value of hai_goods.goods_desc
*
* @mbg.generated Sun May 28 01:02:22 CST 2017
*/
public String getGoodsDesc() {
return goodsDesc;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column hai_goods.goods_desc
*
* @param goodsDesc the value for hai_goods.goods_desc
*
* @mbg.generated Sun May 28 01:02:22 CST 2017
*/
public void setGoodsDesc(String goodsDesc) {
this.goodsDesc = goodsDesc;
}
}<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/mapper/HaiHrCompanyMapper.java
package com.ehais.hrlucene.mapper;
import com.ehais.hrlucene.model.HaiHrCompany;
import com.ehais.hrlucene.model.HaiHrCompanyExample;
import java.util.List;
import org.apache.ibatis.annotations.Param;
public interface HaiHrCompanyMapper {
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
int countByExample(HaiHrCompanyExample example);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
int deleteByExample(HaiHrCompanyExample example);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
int deleteByPrimaryKey(Long company_id);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
int insert(HaiHrCompany record);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
int insertSelective(HaiHrCompany record);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
List<HaiHrCompany> selectByExampleWithBLOBs(HaiHrCompanyExample example);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
List<HaiHrCompany> selectByExample(HaiHrCompanyExample example);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
HaiHrCompany selectByPrimaryKey(Long company_id);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
int updateByExampleSelective(@Param("record") HaiHrCompany record, @Param("example") HaiHrCompanyExample example);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
int updateByExampleWithBLOBs(@Param("record") HaiHrCompany record, @Param("example") HaiHrCompanyExample example);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
int updateByExample(@Param("record") HaiHrCompany record, @Param("example") HaiHrCompanyExample example);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
int updateByPrimaryKeySelective(HaiHrCompany record);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
int updateByPrimaryKeyWithBLOBs(HaiHrCompany record);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table hai_hr_company
* @mbg.generated Sun Nov 27 14:14:10 CST 2016
*/
int updateByPrimaryKey(HaiHrCompany record);
}<file_sep>/reptileV1/src/main/java/com/ehais/window/ShopReptileSWTApplication.java
package com.ehais.window;
import java.util.Map;
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.KeyAdapter;
import org.eclipse.swt.events.KeyEvent;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Group;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
import org.ehais.tools.ReturnObject;
import com.ehais.bean.Goods;
import com.ehais.http.HttpGoods;
import com.ehais.reptile.Reptile;
import com.ehais.reptile.ReptileRunnable;
import com.ehais.reptile.ReptileThread;
import com.ehais.reptile.impl.MytheresaImpl;
import com.ehais.reptile.impl.ShopBopImpl;
public class ShopReptileSWTApplication {
protected Shell shell;
private Text text_weburl;
private Text text_result;
private int url_type;//0:列表,1:内容
private Reptile reptile ;
private ShopBopImpl shopbop;
private MytheresaImpl mytheresa;
private ReturnObject<Object> rm;
private Map<String,String> map;
private HttpGoods httpGoods;
private Goods goods;
private Text txt_category;
private String result;
private ReptileRunnable reptileRunnable;
private ReptileThread reptileThread;
private Display display;
Button button;
private Label lbl_formula;
private Text txt_currency;
private Text txt_exchangeRate;
private Text txt_shipping;
private Text txt_profit;
private int profitType;
public ShopReptileSWTApplication() {
super();
// TODO Auto-generated constructor stub
// shopbop = new ShopBopImpl();
// mytheresa = new MytheresaImpl();
rm = new ReturnObject<Object>();
url_type = 0;
profitType = 0;
httpGoods = new HttpGoods();
goods = new Goods();
display = new Display();
}
/**
* Launch the application.
* @param args
*/
public static void main(String[] args) {
try {
ShopReptileSWTApplication window = new ShopReptileSWTApplication();
window.open();
} catch (Exception e) {
e.printStackTrace();
}
// System.out.println("main");
}
/**
* Open the window.
*/
public void open() {
Display display = Display.getDefault();
createContents();
shell.open();
shell.layout();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
}
public void formulaAction(){
// System.out.println("公式计算");
// lbl_formula.setText("(价格 * 利润 + 运费)*汇率");
if(profitType == 0){
lbl_formula.setText("币种:"+this.txt_currency.getText()+"((价格 * "+this.txt_profit.getText()+" ) + "+this.txt_shipping.getText()+") * "+this.txt_exchangeRate.getText());
}else{
lbl_formula.setText("币种:"+this.txt_currency.getText()+"((价格 + "+this.txt_profit.getText()+" ) + "+this.txt_shipping.getText()+") * "+this.txt_exchangeRate.getText());
}
}
/**
* Create contents of the window.
*/
protected void createContents() {
shell = new Shell();
shell.setSize(625, 442);
shell.setText("商品抓取小工具");
Group group = new Group(shell, SWT.NONE);
group.setText("抓取的网址类型");
group.setBounds(380, 49, 143, 45);
Label lblNewLabel = new Label(shell, SWT.NONE);
lblNewLabel.setBounds(20, 23, 42, 17);
lblNewLabel.setText("网址:");
text_weburl = new Text(shell, SWT.BORDER);
text_weburl.setBounds(68, 20, 481, 23);
text_result = new Text(shell, SWT.BORDER | SWT.H_SCROLL | SWT.V_SCROLL | SWT.CANCEL | SWT.MULTI);
text_result.setBounds(10, 165, 589, 229);
//初始化线程
reptileRunnable = new ReptileRunnable(text_result);
// reptileThread = new ReptileThread(display ,text_result);
// reptileRunnable.setTextResult(text_result);
Label label = new Label(shell, SWT.NONE);
label.setBounds(380, 100, 52, 23);
label.setText("类别:");
txt_category = new Text(shell, SWT.BORDER);
txt_category.setBounds(438, 100, 131, 23);
button = new Button(shell, SWT.NONE);
button.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
String url = text_weburl.getText();
if(url == null || url.equals("")){
return;
}
if(txt_category.getText() == null || txt_category.getText().equals("")){
// JOptionPane.showMessageDialog( null,"请输入分类名称");
// JOptionPane.showMessageDialog(null,"请输入分类名称!!!");
// txt_category.forceFocus();
txt_category.setText("所有分类");
// return;
}
reptileRunnable.setType(url_type);
reptileRunnable.setUrl(url);
reptileRunnable.setCategory_name(txt_category.getText());
reptileRunnable.setCurrency(txt_currency.getText());
reptileRunnable.setExchangeRate(txt_exchangeRate.getText());
reptileRunnable.setProfit(txt_profit.getText());
reptileRunnable.setProfitType(profitType);
reptileRunnable.setShipping(txt_shipping.getText());
//
if(url.toLowerCase().indexOf("shopbop")>0){
new Thread(reptileRunnable, "shopbop").start();
}else if(url.toLowerCase().indexOf("mytheresa")>0){
new Thread(reptileRunnable, "mytheresa").start();
}else if(url.toLowerCase().indexOf("net-a-porter") > 0){
new Thread(reptileRunnable, "net-a-porter").start();
}else if(url.toLowerCase().indexOf("lanecrawford") > 0){
new Thread(reptileRunnable, "lanecrawford").start();
}else if(url.toLowerCase().indexOf("stylebop") > 0){
new Thread(reptileRunnable, "stylebop").start();
}
text_weburl.forceFocus();
}
});
button.setBounds(529, 62, 80, 27);
button.setText("抓取列表");
Button button_1 = new Button(shell, SWT.NONE);
button_1.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
text_weburl.setText("");
text_weburl.setFocus();
}
});
button_1.setBounds(555, 16, 54, 27);
button_1.setText("清空");
Button type_info = new Button(group, SWT.RADIO);
type_info.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
url_type = 1;
button.setText("抓取商品");
}
});
type_info.setBounds(84, 25, 53, 17);
type_info.setText("内容");
Button type_list = new Button(group, SWT.RADIO);
type_list.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
url_type = 0;
button.setText("抓取列表");
}
});
type_list.setSelection(true);
type_list.setBounds(10, 25, 53, 17);
type_list.setText("列表");
txt_category.setVisible(false);
label.setVisible(false);
Group group_1 = new Group(shell, SWT.NONE);
group_1.setText("价格计算:");
group_1.setBounds(10, 49, 355, 110);
lbl_formula = new Label(group_1, SWT.NONE);
lbl_formula.setBounds(0, 83, 345, 17);
lbl_formula.setText("(价格 * 利润 + 运费)*汇率");
txt_currency = new Text(group_1, SWT.BORDER);
txt_currency.addKeyListener(new KeyAdapter() {
@Override
public void keyReleased(KeyEvent e) {
// lbl_formula.setText("币种:"+txt_currency.getText());
formulaAction();
}
});
txt_currency.setText("HKD");
txt_currency.setBounds(35, 18, 45, 23);
Label label_2 = new Label(group_1, SWT.NONE);
label_2.setBounds(0, 21, 29, 17);
label_2.setText("币种");
Label label_3 = new Label(group_1, SWT.NONE);
label_3.setBounds(222, 21, 35, 17);
label_3.setText("运费");
Label lbl_profit = new Label(group_1, SWT.NONE);
lbl_profit.setBounds(0, 55, 35, 17);
lbl_profit.setText("利率");
Label lbl_profitType = new Label(group_1, SWT.NONE);
lbl_profitType.setBounds(134, 55, 61, 17);
lbl_profitType.setText("利润类别");
Label lbl_exchangeRate = new Label(group_1, SWT.NONE);
lbl_exchangeRate.setBounds(86, 21, 35, 17);
lbl_exchangeRate.setText("汇率");
txt_exchangeRate = new Text(group_1, SWT.BORDER);
txt_exchangeRate.addKeyListener(new KeyAdapter() {
@Override
public void keyReleased(KeyEvent e) {
formulaAction();
}
});
txt_exchangeRate.setText("1.0000");
txt_exchangeRate.setBounds(127, 18, 73, 23);
txt_shipping = new Text(group_1, SWT.BORDER);
txt_shipping.addKeyListener(new KeyAdapter() {
@Override
public void keyReleased(KeyEvent e) {
formulaAction();
}
});
txt_shipping.setText("0");
txt_shipping.setBounds(263, 18, 73, 23);
txt_profit = new Text(group_1, SWT.BORDER);
txt_profit.addKeyListener(new KeyAdapter() {
@Override
public void keyReleased(KeyEvent e) {
formulaAction();
}
});
txt_profit.setText("0");
txt_profit.setBounds(35, 52, 73, 23);
Button rdo_profitType_0 = new Button(group_1, SWT.RADIO);
rdo_profitType_0.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
profitType = 0;
formulaAction();
}
});
rdo_profitType_0.setSelection(true);
rdo_profitType_0.setBounds(201, 55, 45, 17);
rdo_profitType_0.setText("率");
Button rdo_profitType_1 = new Button(group_1, SWT.RADIO);
rdo_profitType_1.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
profitType = 1;
formulaAction();
}
});
rdo_profitType_1.setBounds(248, 55, 45, 17);
rdo_profitType_1.setText("值");
//格式化计算公式
formulaAction();
}
}
<file_sep>/FigoShop/getWebkitGhost.py
from ghost import Ghost
ghost = Ghost()
page, extra_resources = ghost.open("http://xiaorui.cc")
assert page.http_status==200 and 'xiaorui' in ghost.content<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/Service/GoodiesAttr.java
package com.ehais.figoarticle.Service;
/**
* Created by stephen on 5/27/17.
*/
public interface GoodiesAttr {
void transfer();
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/MatchesfashionController.java
package com.ehais.figoarticle.controller;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.lang3.StringUtils;
import org.ehais.util.EHtmlUnit;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.model.HaiCategory;
import com.ehais.figoarticle.model.HaiCategoryExample;
import com.ehais.figoarticle.model.HaiGoodsAttr;
import com.ehais.figoarticle.model.HaiGoodsEntity;
import com.ehais.figoarticle.model.HaiGoodsGallery;
import com.ehais.figoarticle.model.HaiGoodsUrl;
import com.ehais.figoarticle.model.HaiGoodsUrlExample;
import com.ehais.figoarticle.model.HaiGoodsWithBLOBs;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
@Controller
@RequestMapping("/matchesfashion")
public class MatchesfashionController extends FigoCommonController{
private static String url = "https://www.matchesfashion.com";
private int websiteId = 12;
@ResponseBody
@RequestMapping("/brand")
public String brand(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
return "";
}
@ResponseBody
@RequestMapping("/category")
public String category(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
this.category(request, url + "/intl/mens");
this.category(request, url + "/intl/womens");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private void category(HttpServletRequest request,String categoryUrl){
String result = "";
try {
// result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), categoryUrl);
// this.fso_write(categoryUrl, result);
// result = PythonUtil.python("D:\\eh-project\\FigoShop\\getAjaxWeb.py", categoryUrl);
// result = FSO.ReadFileName("C:\\Users\\wugang\\Desktop\\my.html");
result = EHtmlUnit.getAjaxPage(categoryUrl);
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(categoryUrl).timeout(10000).get();
List<HaiCategory> list = new ArrayList<HaiCategory>();
Elements nav = doc.select(".main-menu__item");
nav.remove(5);
nav.remove(4);
nav.remove(3);
for (Element element : nav) {
Element parent = element.select(".main-menu__link").first();
String pHref = parent.attr("href");
if (pHref.indexOf("http") < 0 ) pHref = url + pHref;
HaiCategory pCate = new HaiCategory();
pCate.setCatName(parent.text());
pCate.setCategoryUrl(pHref);
pCate.setIsShow(true);
pCate.setWebsiteId(websiteId);
System.out.println(parent.text());
Elements shopitem = element.select(".shop__cols__item");
if (shopitem.hasText()) {
List<HaiCategory> children = new ArrayList<>();
for (Element element1 : shopitem) {
Elements shop = element1.select("h3");
System.out.println("===" + shop.text());
String cHref = element1.select("a").attr("href");
if (cHref.indexOf("http") < 0 ) cHref = url + cHref;
HaiCategory cCate = new HaiCategory();
cCate.setCatName(shop.text());
cCate.setCategoryUrl(cHref);
cCate.setIsShow(true);
cCate.setWebsiteId(websiteId);
children.add(cCate);
Elements aa = element1.select("li>a");
aa.remove(0);
List<HaiCategory> item = new ArrayList<>();
for (Element element2 : aa) {
System.out.println("======" + element2.text());
String iHref = element2.attr("href");
if (iHref.indexOf("http") < 0 ) iHref = url + iHref;
HaiCategory iCate = new HaiCategory();
iCate.setCatName(element2.text());
iCate.setCategoryUrl(iHref);
iCate.setIsShow(true);
iCate.setWebsiteId(websiteId);
item.add(iCate);
}
cCate.setChildren(item);
}
pCate.setChildren(children);
list.add(pCate);
}else {
Elements aa = element.select(".sub_menu").select("a");
List<HaiCategory> children = new ArrayList<>();
for (Element element1 : aa) {
if (element1.attr("href").contains("/intl/products")
|| element1.attr("href").contains("/intl/login")
|| element1.select("p").hasText()
|| element1.select(".main-link").hasText()) continue;
System.out.println("===" + element1.text());
String cHref = element1.select("a").attr("href");
if (cHref.indexOf("http") < 0 ) cHref = url + cHref;
HaiCategory cCate = new HaiCategory();
cCate.setCatName(element1.text());
cCate.setCategoryUrl(cHref);
cCate.setIsShow(true);
cCate.setWebsiteId(websiteId);
children.add(cCate);
}
pCate.setChildren(children);
list.add(pCate);
}
}
System.out.println("==========================================");
System.out.println("==========================================");
JSONArray arr = JSONArray.fromObject(list);
System.out.println(arr.toString());
// System.out.println(element.html());
System.out.println("==========================================");
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/category";
// String api = "http://localhost:8087/api/category";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
}
@ResponseBody
@RequestMapping("/url")
public String url(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
c.andCategoryUrlLike(url+"%");
List<HaiCategory> listCategory = haiCategoryMapper.selectByExample(example);
for (HaiCategory haiCategory : listCategory) {
// System.out.println(haiCategory.getCategoryUrl());
this.goodsUrl(request, haiCategory.getCategoryUrl(), haiCategory.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsUrl(HttpServletRequest request,String goodsurl,Integer catId){
System.out.println("请求地址:" + goodsurl);
String result = "";
try{
// result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
// this.fso_write(goodsurl, result);
// result = PythonUtil.python("D:/workspace_jee/figoarticle/src/main/webapp/getAjaxWeb.py", categoryUrl);
// result = FSO.ReadFileName("E:/temp/IFCHIC.htm");
result = EHtmlUnit.getAjaxPage(goodsurl);
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(goodsurl).timeout(10000).get();
Element productList = doc.select("ul.lister__wrapper").first();
if (productList == null || productList.equals("")) return "";
Elements product_li = productList.select("li.lister__item");
List<String> list = new ArrayList<String>();
for (Element element : product_li) {
String Href = element.select("a").first().attr("href");
if (Href.indexOf("http") < 0) Href = url + Href;
System.out.println(Href);
list.add(Href);
}
JSONArray arr = JSONArray.fromObject(list);
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("catId", catId.toString());
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/url";
// String api = "http://localhost:8087/api/url";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
//获取下一页
Element next = doc.select(".next").first();
if (next != null) {
String nHref = next.select("a").attr("href");
if (nHref.indexOf("http") < 0) nHref = url + nHref;
this.goodsUrl(request, nHref, catId);
}
}catch(Exception e){
e.printStackTrace();
}
return result;
}
@ResponseBody
@RequestMapping("/goodsAll")
public String goodsAll(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiGoodsUrlExample example = new HaiGoodsUrlExample();
HaiGoodsUrlExample.Criteria c = example.createCriteria();
c.andGoodsUrlLike(url+"%");
c.andFinishEqualTo(false);
List<HaiGoodsUrl> listGoodsUrl = haiGoodsUrlMapper.selectByExample(example);
for (HaiGoodsUrl haiGoodsUrl : listGoodsUrl) {
goodsModel(request,haiGoodsUrl.getGoodsUrl(),haiGoodsUrl.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
public String goodsModel(HttpServletRequest request , String goodsurl ,Integer catId){
System.out.println(goodsurl);
String result = "";
HaiGoodsEntity entity = new HaiGoodsEntity();
HaiGoodsWithBLOBs goods = new HaiGoodsWithBLOBs();
List<HaiGoodsGallery> goodsGalleryList = new ArrayList<HaiGoodsGallery>();
List<HaiGoodsAttr> goodsAttrList = new ArrayList<HaiGoodsAttr>();
try{
// result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
result = EHtmlUnit.getAjaxPage(goodsurl);
this.fso_write(goodsurl, result);
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(goodsurl).timeout(10000).get();
Element info = doc.select(".pdp__description-wrapper").first();
String name = info.select(".pdp-description").first().text();
Element priceE = info.select(".pdp-price").first();
String priceS = "";
if (priceE.select(".pdp-price__hilite").size() != 0) {
Element price2 = priceE.select(".pdp-price__hilite").get(1);
priceS = price2.text().split("/ ")[1];
} else {
priceS = priceE.text().split("/ ")[1];
}
priceS = priceS.replace(",","");
Integer price = Float.valueOf(priceS.substring(1)).intValue() * 100;
String currency = priceS.substring(0,1);
String desc = doc.select(".scroller-content").first().text() + " " + doc.select(".pdp-accordion__body__details-list").first().text();
goods.setGoodsName(name);
goods.setGoodsDesc(desc);
goods.setCurrency(currency);
goods.setShopPrice(price);
goods.setGoodsUrl(goodsurl);
goods.setCatId(catId);
goods.setWebsiteId(websiteId);
Elements slick_track = doc.getElementsByClass("slick-track");
for (Element element : slick_track) {
Elements ediv = element.select(">div");
for (Element element2 : ediv) {
String imgstr = element2.attr("data-main-img-url");
if(StringUtils.isNotBlank(imgstr)){
String gHref = "http:" + imgstr;
HaiGoodsGallery gallery = new HaiGoodsGallery();
gallery.setThumbUrl(gHref);
gallery.setImgUrl(gHref);
gallery.setImgOriginal(gHref);
goodsGalleryList.add(gallery);
}
}
}
//picture
// Elements gallery_ul = doc.select(".thumbs-gallery__thumb");
// Elements gallery_li = gallery_ul.select("img");
// for (Element element : gallery_li) {
// String gHref = "http:" + element.attr("src");
// HaiGoodsGallery gallery = new HaiGoodsGallery();
// gallery.setThumbUrl(gHref);
// gallery.setImgUrl(gHref);
// gallery.setImgOriginal(gHref);
// goodsGalleryList.add(gallery);
// }
if(goodsGalleryList.size() > 0){
HaiGoodsGallery gallery = goodsGalleryList.get(0);
goods.setGoodsThumb(gallery.getThumbUrl());
goods.setGoodsImg(gallery.getImgUrl());
goods.setOriginalImg(gallery.getImgOriginal());
}
//size
Elements size = doc.select(".sg__table");
if (size.isEmpty()) {
HaiGoodsAttr goodsSize = new HaiGoodsAttr();
goodsSize.setAttrValue("One Size");
goodsSize.setAttrType("size");
goodsSize.setAttrPrice(goods.getShopPrice().toString());
goodsAttrList.add(goodsSize);
}else {
Elements sizespan = size.select(".sg__table-cell");
sizespan.remove(0);
List<String> sizeList = new ArrayList<>();
for (Element element : sizespan) {
HaiGoodsAttr goodsSize = new HaiGoodsAttr();
goodsSize.setAttrValue(element.text());
goodsSize.setAttrType("size");
goodsSize.setAttrPrice(goods.getShopPrice().toString());
sizeList.add(element.text());
goodsAttrList.add(goodsSize);
}
}
// Bean2Utils.printEntity(goods);
entity.setGoods(goods);
entity.setGoodsAttrList(goodsAttrList);
entity.setGoodsGalleryList(goodsGalleryList);
JSONObject jsonObject = JSONObject.fromObject(entity);
System.out.println(jsonObject.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", jsonObject.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/goodsAttr";
// String api = "http://localhost:8087/api/goods";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
public static void main(String[] args) throws Exception {
String goodsurl = "https://www.net-a-porter.com/cn/zh/d/Shop/Lingerie/All?cm_sp=topnav-_-clothing-_-lingerie";
DemoController ac = new DemoController();
// ac.goodsModel(url,1);
// ac.goodsUrl(null, goodsurl, 1);
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/mapper/HaiGoodsGalleryMapper.java
package com.ehais.figoarticle.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import com.ehais.figoarticle.model.HaiGoodsGallery;
import com.ehais.figoarticle.model.HaiGoodsGalleryExample;
public interface HaiGoodsGalleryMapper {
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_gallery
*
* @mbg.generated Fri Apr 14 21:52:06 CST 2017
*/
long countByExample(HaiGoodsGalleryExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_gallery
*
* @mbg.generated Fri Apr 14 21:52:06 CST 2017
*/
int deleteByExample(HaiGoodsGalleryExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_gallery
*
* @mbg.generated Fri Apr 14 21:52:06 CST 2017
*/
int deleteByPrimaryKey(Long imgId);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_gallery
*
* @mbg.generated Fri Apr 14 21:52:06 CST 2017
*/
int insert(HaiGoodsGallery record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_gallery
*
* @mbg.generated Fri Apr 14 21:52:06 CST 2017
*/
int insertSelective(HaiGoodsGallery record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_gallery
*
* @mbg.generated Fri Apr 14 21:52:06 CST 2017
*/
List<HaiGoodsGallery> selectByExample(HaiGoodsGalleryExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_gallery
*
* @mbg.generated Fri Apr 14 21:52:06 CST 2017
*/
HaiGoodsGallery selectByPrimaryKey(Long imgId);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_gallery
*
* @mbg.generated Fri Apr 14 21:52:06 CST 2017
*/
int updateByExampleSelective(@Param("record") HaiGoodsGallery record, @Param("example") HaiGoodsGalleryExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_gallery
*
* @mbg.generated Fri Apr 14 21:52:06 CST 2017
*/
int updateByExample(@Param("record") HaiGoodsGallery record, @Param("example") HaiGoodsGalleryExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_gallery
*
* @mbg.generated Fri Apr 14 21:52:06 CST 2017
*/
int updateByPrimaryKeySelective(HaiGoodsGallery record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table hai_goods_gallery
*
* @mbg.generated Fri Apr 14 21:52:06 CST 2017
*/
int updateByPrimaryKey(HaiGoodsGallery record);
}<file_sep>/eh-weixin/src/main/java/org/ehais/weixin/mapper/WxNotityPayMapper.java
package org.ehais.weixin.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import org.ehais.weixin.model.WxNotityPay;
import org.ehais.weixin.model.WxNotityPayExample;
public interface WxNotityPayMapper {
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_notity_pay
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
int countByExample(WxNotityPayExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_notity_pay
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
int deleteByExample(WxNotityPayExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_notity_pay
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
int deleteByPrimaryKey(Integer notifyPayId);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_notity_pay
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
int insert(WxNotityPay record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_notity_pay
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
int insertSelective(WxNotityPay record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_notity_pay
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
List<WxNotityPay> selectByExample(WxNotityPayExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_notity_pay
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
WxNotityPay selectByPrimaryKey(Integer notifyPayId);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_notity_pay
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
int updateByExampleSelective(@Param("record") WxNotityPay record, @Param("example") WxNotityPayExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_notity_pay
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
int updateByExample(@Param("record") WxNotityPay record, @Param("example") WxNotityPayExample example);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_notity_pay
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
int updateByPrimaryKeySelective(WxNotityPay record);
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table wx_notity_pay
*
* @mbggenerated Fri Feb 26 21:48:08 CST 2016
*/
int updateByPrimaryKey(WxNotityPay record);
}<file_sep>/school-weixin/src/main/java/com/ehais/schoolweixin/model/activitynameExample.java
package com.ehais.schoolweixin.model;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class activitynameExample {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
protected String orderByClause;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
protected boolean distinct;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
protected List<Criteria> oredCriteria;
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
public activitynameExample() {
oredCriteria = new ArrayList<Criteria>();
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
public String getOrderByClause() {
return orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
public boolean isDistinct() {
return distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andIdIsNull() {
addCriterion("id is null");
return (Criteria) this;
}
public Criteria andIdIsNotNull() {
addCriterion("id is not null");
return (Criteria) this;
}
public Criteria andIdEqualTo(Integer value) {
addCriterion("id =", value, "id");
return (Criteria) this;
}
public Criteria andIdNotEqualTo(Integer value) {
addCriterion("id <>", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThan(Integer value) {
addCriterion("id >", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThanOrEqualTo(Integer value) {
addCriterion("id >=", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThan(Integer value) {
addCriterion("id <", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThanOrEqualTo(Integer value) {
addCriterion("id <=", value, "id");
return (Criteria) this;
}
public Criteria andIdIn(List<Integer> values) {
addCriterion("id in", values, "id");
return (Criteria) this;
}
public Criteria andIdNotIn(List<Integer> values) {
addCriterion("id not in", values, "id");
return (Criteria) this;
}
public Criteria andIdBetween(Integer value1, Integer value2) {
addCriterion("id between", value1, value2, "id");
return (Criteria) this;
}
public Criteria andIdNotBetween(Integer value1, Integer value2) {
addCriterion("id not between", value1, value2, "id");
return (Criteria) this;
}
public Criteria andActivityNameIsNull() {
addCriterion("activityName is null");
return (Criteria) this;
}
public Criteria andActivityNameIsNotNull() {
addCriterion("activityName is not null");
return (Criteria) this;
}
public Criteria andActivityNameEqualTo(String value) {
addCriterion("activityName =", value, "activityName");
return (Criteria) this;
}
public Criteria andActivityNameNotEqualTo(String value) {
addCriterion("activityName <>", value, "activityName");
return (Criteria) this;
}
public Criteria andActivityNameGreaterThan(String value) {
addCriterion("activityName >", value, "activityName");
return (Criteria) this;
}
public Criteria andActivityNameGreaterThanOrEqualTo(String value) {
addCriterion("activityName >=", value, "activityName");
return (Criteria) this;
}
public Criteria andActivityNameLessThan(String value) {
addCriterion("activityName <", value, "activityName");
return (Criteria) this;
}
public Criteria andActivityNameLessThanOrEqualTo(String value) {
addCriterion("activityName <=", value, "activityName");
return (Criteria) this;
}
public Criteria andActivityNameLike(String value) {
addCriterion("activityName like", value, "activityName");
return (Criteria) this;
}
public Criteria andActivityNameNotLike(String value) {
addCriterion("activityName not like", value, "activityName");
return (Criteria) this;
}
public Criteria andActivityNameIn(List<String> values) {
addCriterion("activityName in", values, "activityName");
return (Criteria) this;
}
public Criteria andActivityNameNotIn(List<String> values) {
addCriterion("activityName not in", values, "activityName");
return (Criteria) this;
}
public Criteria andActivityNameBetween(String value1, String value2) {
addCriterion("activityName between", value1, value2, "activityName");
return (Criteria) this;
}
public Criteria andActivityNameNotBetween(String value1, String value2) {
addCriterion("activityName not between", value1, value2, "activityName");
return (Criteria) this;
}
public Criteria andPCountIsNull() {
addCriterion("pCount is null");
return (Criteria) this;
}
public Criteria andPCountIsNotNull() {
addCriterion("pCount is not null");
return (Criteria) this;
}
public Criteria andPCountEqualTo(Integer value) {
addCriterion("pCount =", value, "pCount");
return (Criteria) this;
}
public Criteria andPCountNotEqualTo(Integer value) {
addCriterion("pCount <>", value, "pCount");
return (Criteria) this;
}
public Criteria andPCountGreaterThan(Integer value) {
addCriterion("pCount >", value, "pCount");
return (Criteria) this;
}
public Criteria andPCountGreaterThanOrEqualTo(Integer value) {
addCriterion("pCount >=", value, "pCount");
return (Criteria) this;
}
public Criteria andPCountLessThan(Integer value) {
addCriterion("pCount <", value, "pCount");
return (Criteria) this;
}
public Criteria andPCountLessThanOrEqualTo(Integer value) {
addCriterion("pCount <=", value, "pCount");
return (Criteria) this;
}
public Criteria andPCountIn(List<Integer> values) {
addCriterion("pCount in", values, "pCount");
return (Criteria) this;
}
public Criteria andPCountNotIn(List<Integer> values) {
addCriterion("pCount not in", values, "pCount");
return (Criteria) this;
}
public Criteria andPCountBetween(Integer value1, Integer value2) {
addCriterion("pCount between", value1, value2, "pCount");
return (Criteria) this;
}
public Criteria andPCountNotBetween(Integer value1, Integer value2) {
addCriterion("pCount not between", value1, value2, "pCount");
return (Criteria) this;
}
public Criteria andStarTimeIsNull() {
addCriterion("starTime is null");
return (Criteria) this;
}
public Criteria andStarTimeIsNotNull() {
addCriterion("starTime is not null");
return (Criteria) this;
}
public Criteria andStarTimeEqualTo(Date value) {
addCriterion("starTime =", value, "starTime");
return (Criteria) this;
}
public Criteria andStarTimeNotEqualTo(Date value) {
addCriterion("starTime <>", value, "starTime");
return (Criteria) this;
}
public Criteria andStarTimeGreaterThan(Date value) {
addCriterion("starTime >", value, "starTime");
return (Criteria) this;
}
public Criteria andStarTimeGreaterThanOrEqualTo(Date value) {
addCriterion("starTime >=", value, "starTime");
return (Criteria) this;
}
public Criteria andStarTimeLessThan(Date value) {
addCriterion("starTime <", value, "starTime");
return (Criteria) this;
}
public Criteria andStarTimeLessThanOrEqualTo(Date value) {
addCriterion("starTime <=", value, "starTime");
return (Criteria) this;
}
public Criteria andStarTimeIn(List<Date> values) {
addCriterion("starTime in", values, "starTime");
return (Criteria) this;
}
public Criteria andStarTimeNotIn(List<Date> values) {
addCriterion("starTime not in", values, "starTime");
return (Criteria) this;
}
public Criteria andStarTimeBetween(Date value1, Date value2) {
addCriterion("starTime between", value1, value2, "starTime");
return (Criteria) this;
}
public Criteria andStarTimeNotBetween(Date value1, Date value2) {
addCriterion("starTime not between", value1, value2, "starTime");
return (Criteria) this;
}
public Criteria andEndTimeIsNull() {
addCriterion("endTime is null");
return (Criteria) this;
}
public Criteria andEndTimeIsNotNull() {
addCriterion("endTime is not null");
return (Criteria) this;
}
public Criteria andEndTimeEqualTo(Date value) {
addCriterion("endTime =", value, "endTime");
return (Criteria) this;
}
public Criteria andEndTimeNotEqualTo(Date value) {
addCriterion("endTime <>", value, "endTime");
return (Criteria) this;
}
public Criteria andEndTimeGreaterThan(Date value) {
addCriterion("endTime >", value, "endTime");
return (Criteria) this;
}
public Criteria andEndTimeGreaterThanOrEqualTo(Date value) {
addCriterion("endTime >=", value, "endTime");
return (Criteria) this;
}
public Criteria andEndTimeLessThan(Date value) {
addCriterion("endTime <", value, "endTime");
return (Criteria) this;
}
public Criteria andEndTimeLessThanOrEqualTo(Date value) {
addCriterion("endTime <=", value, "endTime");
return (Criteria) this;
}
public Criteria andEndTimeIn(List<Date> values) {
addCriterion("endTime in", values, "endTime");
return (Criteria) this;
}
public Criteria andEndTimeNotIn(List<Date> values) {
addCriterion("endTime not in", values, "endTime");
return (Criteria) this;
}
public Criteria andEndTimeBetween(Date value1, Date value2) {
addCriterion("endTime between", value1, value2, "endTime");
return (Criteria) this;
}
public Criteria andEndTimeNotBetween(Date value1, Date value2) {
addCriterion("endTime not between", value1, value2, "endTime");
return (Criteria) this;
}
public Criteria andFinalTimeIsNull() {
addCriterion("finalTime is null");
return (Criteria) this;
}
public Criteria andFinalTimeIsNotNull() {
addCriterion("finalTime is not null");
return (Criteria) this;
}
public Criteria andFinalTimeEqualTo(Date value) {
addCriterion("finalTime =", value, "finalTime");
return (Criteria) this;
}
public Criteria andFinalTimeNotEqualTo(Date value) {
addCriterion("finalTime <>", value, "finalTime");
return (Criteria) this;
}
public Criteria andFinalTimeGreaterThan(Date value) {
addCriterion("finalTime >", value, "finalTime");
return (Criteria) this;
}
public Criteria andFinalTimeGreaterThanOrEqualTo(Date value) {
addCriterion("finalTime >=", value, "finalTime");
return (Criteria) this;
}
public Criteria andFinalTimeLessThan(Date value) {
addCriterion("finalTime <", value, "finalTime");
return (Criteria) this;
}
public Criteria andFinalTimeLessThanOrEqualTo(Date value) {
addCriterion("finalTime <=", value, "finalTime");
return (Criteria) this;
}
public Criteria andFinalTimeIn(List<Date> values) {
addCriterion("finalTime in", values, "finalTime");
return (Criteria) this;
}
public Criteria andFinalTimeNotIn(List<Date> values) {
addCriterion("finalTime not in", values, "finalTime");
return (Criteria) this;
}
public Criteria andFinalTimeBetween(Date value1, Date value2) {
addCriterion("finalTime between", value1, value2, "finalTime");
return (Criteria) this;
}
public Criteria andFinalTimeNotBetween(Date value1, Date value2) {
addCriterion("finalTime not between", value1, value2, "finalTime");
return (Criteria) this;
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table activityname
*
* @mbggenerated do_not_delete_during_merge Sun Feb 26 23:05:24 CST 2017
*/
public static class Criteria extends GeneratedCriteria {
protected Criteria() {
super();
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table activityname
*
* @mbggenerated Sun Feb 26 23:05:24 CST 2017
*/
public static class Criterion {
private String condition;
private Object value;
private Object secondValue;
private boolean noValue;
private boolean singleValue;
private boolean betweenValue;
private boolean listValue;
private String typeHandler;
public String getCondition() {
return condition;
}
public Object getValue() {
return value;
}
public Object getSecondValue() {
return secondValue;
}
public boolean isNoValue() {
return noValue;
}
public boolean isSingleValue() {
return singleValue;
}
public boolean isBetweenValue() {
return betweenValue;
}
public boolean isListValue() {
return listValue;
}
public String getTypeHandler() {
return typeHandler;
}
protected Criterion(String condition) {
super();
this.condition = condition;
this.typeHandler = null;
this.noValue = true;
}
protected Criterion(String condition, Object value, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.typeHandler = typeHandler;
if (value instanceof List<?>) {
this.listValue = true;
} else {
this.singleValue = true;
}
}
protected Criterion(String condition, Object value) {
this(condition, value, null);
}
protected Criterion(String condition, Object value, Object secondValue, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.secondValue = secondValue;
this.typeHandler = typeHandler;
this.betweenValue = true;
}
protected Criterion(String condition, Object value, Object secondValue) {
this(condition, value, secondValue, null);
}
}
}<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/FwrdController.java
package com.ehais.figoarticle.controller;
import com.ehais.figoarticle.model.*;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
//完成
@Controller
@RequestMapping("/fwrd")
public class FwrdController extends FigoCommonController{
private static String url = "http://www.fwrd.com";
private int websiteId = 6;
@ResponseBody
@RequestMapping("/brand")
public String brand(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
return "";
}
@ResponseBody
@RequestMapping("/category")
public String category(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
this.category(request, url + "/?navsrc=global");
this.category(request,url + "/?d=Mens&navsrc=global");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private void category(HttpServletRequest request,String categoryUrl){
System.out.println("请求地址:" + categoryUrl);
String result = "";
// Connection.Response response = null;
try {
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), categoryUrl);
// result = PythonUtil.python("E:\\code\\eh-project\\FigoShop\\getEnWebForFwrd.py", categoryUrl);
// response = Jsoup.connect(categoryUrl).execute();
System.out.println(result);
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(categoryUrl).get();
List<HaiCategory> list = new ArrayList<HaiCategory>();
Element sex = doc.select(".current").first();
System.out.println(sex.text());
Element sex_a = sex.select("a").first();
String sexHref = sex_a.attr("href");
if (sexHref.indexOf("http") < 0) sexHref = url + sexHref;
HaiCategory topcat = new HaiCategory();
topcat.setCatName(sex.text());
topcat.setCategoryUrl(sexHref);
topcat.setIsShow(true);
topcat.setWebsiteId(websiteId);
List<HaiCategory> topcatlist = new ArrayList<HaiCategory>();
Elements pageitem = doc.select(".page_item");
for (Element element1 : pageitem) {
Element item_a = element1.select("a").first();
String itemHref = item_a.attr("href");
System.out.println("===" + item_a.text());
if (itemHref.indexOf("http") < 0 ) itemHref = url + itemHref;
HaiCategory cat2 = new HaiCategory();
cat2.setCatName(item_a.text());
cat2.setCategoryUrl(itemHref);
cat2.setIsShow(true);
cat2.setWebsiteId(websiteId);
topcatlist.add(cat2);
Element sub_menu = element1.select(".sub_menu").first();
if ( sub_menu != null ) {
List<HaiCategory> catlist = new ArrayList<HaiCategory>();
Elements taga = sub_menu.select(".spread");
if (taga.size() == 0) {
taga = sub_menu.select("li").not("[style^=display]");
taga = taga.select("a");
} else {
taga = sub_menu.select("a.u-padding-a--none");
}
for(Element element2 : taga) {
String aName = element2.text();
System.out.println("======" + aName);
String aHref = element2.attr("href");
if (aHref.indexOf("http") < 0) aHref = url + aHref;
HaiCategory cat3 = new HaiCategory();
cat3.setCatName(aName);
cat3.setCategoryUrl(aHref);
cat3.setIsShow(true);
cat3.setWebsiteId(websiteId);
catlist.add(cat3);
}
cat2.setChildren(catlist);
}
}
topcat.setChildren(topcatlist);
list.add(topcat);
System.out.println("==========================================");
System.out.println("==========================================");
JSONArray arr = JSONArray.fromObject(list);
System.out.println(arr.toString());
// System.out.println(element.html());
System.out.println("==========================================");
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/category";
// String api = "http://localhost:8087/api/category";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
}
@ResponseBody
@RequestMapping("/url")
public String url(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
c.andCategoryUrlLike(url+"%");
List<HaiCategory> listCategory = haiCategoryMapper.selectByExample(example);
for (HaiCategory haiCategory : listCategory) {
System.out.println(haiCategory.getCategoryUrl());
this.goodsUrl(request, haiCategory.getCategoryUrl(), haiCategory.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsUrl(HttpServletRequest request,String goodsurl,Integer catId){
System.out.println("请求地址:"+ goodsurl);
String result = "";
try{
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
// result = PythonUtil.python("D:/workspace_jee/figoarticle/src/main/webapp/getAjaxWeb.py", categoryUrl);
// result = FSO.ReadFileName("E:/temp/IFCHIC.htm");
Document doc = Jsoup.parse(result);
// Document doc = Jsoup.connect(goodsurl).get();
List<String> list = new ArrayList<String>();
Elements page = doc.select(".pagination__list");
if (page.isEmpty()) return "";
int lastpage = Integer.parseInt(page.select("li").last().text().toString());
for (int i = 1; i <= lastpage; i++) {
if (lastpage != 1 ) {
doc = Jsoup.connect(goodsurl + "&pageNum=" + i).get();
}
Elements productlist = doc.select(".product__link");
if (productlist == null) {
return "";
}
for (Element element : productlist) {
String productHref = element.attr("href");
if (element.attr("href").indexOf("http") < 0) productHref = url + productHref;
System.out.println(productHref);
list.add(productHref);
}
}
JSONArray arr = JSONArray.fromObject(list);
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("catId", catId.toString());
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/url";
// String api = "http://localhost:8087/api/url";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
//获取下一页
/**
Element page_chooser = page.getElementById("page-chooser");
Element next = page_chooser.getElementsByClass("next").first();
if(next != null){
String href_a = next.attr("href");
this.goodsUrl(request, href_a, catId);
}
**/
}catch(Exception e){
e.printStackTrace();
}
return result;
}
@ResponseBody
@RequestMapping("/goodsAll")
public String goodsAll(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiGoodsUrlExample example = new HaiGoodsUrlExample();
HaiGoodsUrlExample.Criteria c = example.createCriteria();
c.andGoodsUrlLike(url+"%");
c.andFinishEqualTo(false);
List<HaiGoodsUrl> listGoodsUrl = haiGoodsUrlMapper.selectByExample(example);
for (HaiGoodsUrl haiGoodsUrl : listGoodsUrl) {
goodsModel(request,haiGoodsUrl.getGoodsUrl(),haiGoodsUrl.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
public String goodsModel(HttpServletRequest request , String goodsurl ,Integer catId){
System.out.println("请求地址:" + goodsurl);
String result = "";
HaiGoodsEntity entity = new HaiGoodsEntity();
HaiGoodsWithBLOBs goods = new HaiGoodsWithBLOBs();
List<HaiGoodsGallery> goodsGalleryList = new ArrayList<HaiGoodsGallery>();
List<HaiGoodsAttr> goodsAttrList = new ArrayList<HaiGoodsAttr>();
goods.setGoodsUrl(goodsurl);
goods.setCatId(catId);
goods.setWebsiteId(websiteId);
try{
// Document doc = Jsoup.connect(goodsurl).timeout(10000).get();
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
Document doc = Jsoup.parse(result);
Element info = doc.select(".product_info").first();
String name = info.select(".product-titles__brand").text();
String priceS = "";
Element priceE = info.select("#tr-pdp-price").first();
if ( info.select("#tr-pdp-price").isEmpty() ) {
priceS = info.select(".prices__markdown").first().text().substring(3);
} else {
priceS = priceE.select(".prices_retail").text().substring(3);
}
String priceL = priceS.replace(",","");
Integer price = Float.valueOf(priceL).intValue() * 100;
String currency = doc.select(".currency_select").select("a").first().text();
String desc = info.select("#details").text();
goods.setGoodsName(name);
goods.setShopPrice(price);
goods.setGoodsDesc(desc);
goods.setCurrency(currency);
//picture
Elements gallery_ul = doc.select("img.product-detail-image");
for (Element element : gallery_ul) {
String gHref = element.attr("data-zoom-image");
HaiGoodsGallery gallery = new HaiGoodsGallery();
gallery.setImgOriginal(gHref);
gallery.setImgUrl(gHref);
gallery.setThumbUrl(gHref);
goodsGalleryList.add(gallery);
}
HaiGoodsGallery gallery = goodsGalleryList.get(0);
goods.setGoodsThumb(gallery.getThumbUrl());
goods.setGoodsImg(gallery.getImgUrl());
goods.setOriginalImg(gallery.getImgOriginal());
//color
Element color = doc.select(".color_dd").first();
if ( !color.select(".one_sizeonly").isEmpty() ) {
HaiGoodsAttr goodsColor = new HaiGoodsAttr();
goodsColor.setAttrType("color");
goodsColor.setAttrValue(color.select(".one_sizeonly").text());
goodsColor.setAttrPrice(price.toString());
goodsAttrList.add(goodsColor);
} else {
Element colors = doc.select("#color-select").first();
Elements coloroption = colors.select("option");
coloroption.remove(0);
for (Element element : coloroption) {
HaiGoodsAttr goodsColor = new HaiGoodsAttr();
goodsColor.setAttrValue(element.attr("value"));
goodsColor.setAttrType("color");
goodsColor.setAttrPrice(price.toString());
goodsAttrList.add(goodsColor);
}
}
//size
Element size = doc.select(".size_dd").first();
if ( !size.select("#one-size-div").isEmpty() ) {
HaiGoodsAttr goodsSize = new HaiGoodsAttr();
goodsSize.setAttrType("size");
goodsSize.setAttrValue(size.select("#one-size-div").text());
goodsSize.setAttrPrice(price.toString());
} else {
Element sizes = doc.select("#size-select").first();
Elements sizeoption = sizes.select("option");
sizeoption.remove(0);
for ( Element element : sizeoption) {
HaiGoodsAttr goodsSize = new HaiGoodsAttr();
goodsSize.setAttrValue(element.attr("value"));
goodsSize.setAttrType("size");
goodsSize.setAttrPrice(price.toString());
goodsAttrList.add(goodsSize);
}
}
// Bean2Utils.printEntity(goods);
entity.setGoods(goods);
entity.setGoodsAttrList(goodsAttrList);
entity.setGoodsGalleryList(goodsGalleryList);
JSONObject jsonObject = JSONObject.fromObject(entity);
System.out.println(jsonObject.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", jsonObject.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/goodsAttr";
// String api = "http://localhost:8087/api/goods";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
//单商品的地址请求
@ResponseBody
@RequestMapping("/getGoodsUrl")
public String getGoodsUrl(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "catId", required = true) Integer catId,
@RequestParam(value = "goodsurl", required = true) String goodsurl
){
try{
return goodsModel(request,goodsurl,catId);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
public static void main(String[] args) throws Exception {
String goodsurl = "https://www.net-a-porter.com/cn/zh/d/Shop/Lingerie/All?cm_sp=topnav-_-clothing-_-lingerie";
DemoController ac = new DemoController();
// ac.goodsModel(url,1);
// ac.goodsUrl(null, goodsurl, 1);
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/NetAPorterController.java
package com.ehais.figoarticle.controller;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.lang3.StringEscapeUtils;
import org.apache.commons.logging.LogFactory;
import org.ehais.controller.CommonController;
import org.ehais.util.Bean2Utils;
import org.ehais.util.EHtmlUnit;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.mapper.HaiBrandMapper;
import com.ehais.figoarticle.mapper.HaiCategoryMapper;
import com.ehais.figoarticle.mapper.HaiGoodsMapper;
import com.ehais.figoarticle.mapper.HaiGoodsUrlMapper;
import com.ehais.figoarticle.model.HaiCategory;
import com.ehais.figoarticle.model.HaiCategoryExample;
import com.ehais.figoarticle.model.HaiGoodsAttr;
import com.ehais.figoarticle.model.HaiGoodsEntity;
import com.ehais.figoarticle.model.HaiGoodsGallery;
import com.ehais.figoarticle.model.HaiGoodsUrl;
import com.ehais.figoarticle.model.HaiGoodsUrlExample;
import com.ehais.figoarticle.model.HaiGoodsWithBLOBs;
import com.gargoylesoftware.htmlunit.BrowserVersion;
import com.gargoylesoftware.htmlunit.NicelyResynchronizingAjaxController;
import com.gargoylesoftware.htmlunit.WebClient;
import com.gargoylesoftware.htmlunit.html.HtmlPage;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
@Controller
@RequestMapping("/net-a-porter")
public class NetAPorterController extends FigoCommonController {
private static String url = "https://www.net-a-porter.com/";
private int websiteId = 15;
@ResponseBody
@RequestMapping("/brand")
public String brand(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
System.out.println(request.getRealPath("/getAjaxWeb.py"));
return "";
}
@ResponseBody
@RequestMapping("/category")
public String category(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
String result = "";
try {
// result = EHtmlUnit.getAjaxPage(url+"cn/zh/");
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), url+"cn/zh/");
Document doc = Jsoup.parse(result);
Element nav_links = doc.getElementsByClass("sf-nav__bar").first();
Elements nav_links_li = nav_links.select(">li");
List<HaiCategory> list = new ArrayList<HaiCategory>();
for (Element element : nav_links_li) {
HaiCategory cat = new HaiCategory();
Map<String, Object> map = new HashMap<String,Object>();
Element ap = element.select("a").first();
cat.setCatName(ap.text());
cat.setCategoryUrl(url+ap.attr("href"));
cat.setIsShow(true);
cat.setWebsiteId(websiteId);
List<HaiCategory> catList = new ArrayList<HaiCategory>();
Elements section = element
.select(".sf-nav__level2").first()
.select(".sf-nav__content").first()
.select(".sf-nav__categories").first()
.select(".sf-nav__section");
for (Element element3 : section) {
Elements li = element3.getElementsByTag("ul").first().getElementsByTag("li");
for (Element element2 : li) {
Element ea = element2.getElementsByTag("a").first();
HaiCategory cat2 = new HaiCategory();
cat2.setCatName(ea.text());
cat2.setCategoryUrl(url+ea.attr("href"));
cat2.setIsShow(true);
cat2.setWebsiteId(websiteId);
catList.add(cat2);
}
cat.setChildren(catList);
}
map.put("catList", catList);
list.add(cat);
}
JSONArray arr = JSONArray.fromObject(list);
System.out.println(arr.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/category";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
System.out.println(apiresult);
return result;
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
@ResponseBody
@RequestMapping("/url")
public String url(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
c.andCategoryUrlLike(url+"%");
List<HaiCategory> listCategory = haiCategoryMapper.selectByExample(example);
for (HaiCategory haiCategory : listCategory) {
this.goodsUrl(request, haiCategory.getCategoryUrl(), haiCategory.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsUrl(HttpServletRequest request,String goodsurl,Integer catId){
System.out.println(goodsurl);
String result = "";
try{
// result = EHtmlUnit.getAjaxPage(goodsurl);
// result = EHttpClientUtil.methodGet(goodsurl);
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
System.out.println(result);
Document doc = Jsoup.parse(result);
Element product_list = doc.getElementById("product-list");
Elements product_li = product_list.getElementsByTag("li");
List<String> list = new ArrayList<String>();
int i = 1;
for (Element element : product_li) {
Element aLi = element.getElementsByTag("a").first();
list.add(url + aLi.attr("href"));
System.out.println(url + aLi.attr("href"));
i++;
}
JSONArray arr = JSONArray.fromObject(list);
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("catId", catId.toString());
paramsMap.put("json", arr.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/url";
// String api = "http://localhost:8087/api/url";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
//获取下一页
String nextUrl = doc.getElementsByClass("next-page").first().attr("href");
nextUrl = nextUrl.replace("../", "");
String[] nextSplit = nextUrl.split("\\?");
goodsurl = goodsurl.substring(0, goodsurl.indexOf(nextSplit[0])) ;
this.goodsUrl(request , goodsurl,catId);
}catch(Exception e){
e.printStackTrace();
}
return result;
}
@ResponseBody
@RequestMapping("/goodsAll")
public String goodsAll(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
try {
HaiGoodsUrlExample example = new HaiGoodsUrlExample();
HaiGoodsUrlExample.Criteria c = example.createCriteria();
c.andGoodsUrlLike(url+"%");
c.andFinishEqualTo(false);
List<HaiGoodsUrl> listGoodsUrl = haiGoodsUrlMapper.selectByExample(example);
for (HaiGoodsUrl haiGoodsUrl : listGoodsUrl) {
goodsModel(request,haiGoodsUrl.getGoodsUrl(),haiGoodsUrl.getCatId());
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return "";
}
private String goodsModel(HttpServletRequest request , String goodsurl ,Integer catId){
System.out.println(goodsurl);
String result = "";
HaiGoodsEntity entity = new HaiGoodsEntity();
HaiGoodsWithBLOBs goods = new HaiGoodsWithBLOBs();
List<HaiGoodsGallery> goodsGalleryList = new ArrayList<HaiGoodsGallery>();
List<HaiGoodsAttr> goodsAttrList = new ArrayList<HaiGoodsAttr>();
try{
result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), goodsurl);
// this.fso_write(goodsurl, result);
Document doc = Jsoup.parse(result);
Element main_product = doc.getElementById("main-product");
String goodsName = main_product.getElementsByClass("product-name").text();
goods.setGoodsName(goodsName);
goods.setCatId(catId);
goods.setWebsiteId(websiteId);
Element product_price = main_product.getElementsByClass("product-price").first();
String product_price_json = StringEscapeUtils.unescapeJava(product_price.attr("price")) ;
product_price_json = product_price_json.replaceAll(""", "\"") ;
JSONObject json = JSONObject.fromObject(product_price_json);
Integer shopPrice = json.getInt("amount");
String currency = json.getString("currency");
goods.setShopPrice(shopPrice);
goods.setCurrency(currency);
HaiGoodsAttr goodsAttr = new HaiGoodsAttr();
Element accordion1 = main_product.getElementById("accordion-1").getElementsByClass("wrapper").first();
String attrValue = accordion1.html();
goodsAttr.setAttrValue(attrValue);
goodsAttr.setAttrPrice(shopPrice.toString());
goodsAttr.setAttrType("text");
goodsAttrList.add(goodsAttr);
Element accordion2 = main_product.getElementById("accordion-2").getElementsByClass("wrapper").first();
String goodsDesc = accordion2.html();
goods.setGoodsDesc(goodsDesc);
goods.setGoodsUrl(goodsurl);
Element main_image_carousel = doc.getElementById("main-image-carousel");
Elements img_list = main_image_carousel.getElementsByTag("li");
String img = "";
for (Element element : img_list) {
img = element.getElementsByTag("img").attr("src");
if(img.indexOf("http")<0)img = "https:"+img;
HaiGoodsGallery gallery = new HaiGoodsGallery();
gallery.setThumbUrl(img);
gallery.setImgUrl(img);
gallery.setImgOriginal(img);
goodsGalleryList.add(gallery);
}
if(goodsGalleryList.size() > 0){
HaiGoodsGallery gallery = goodsGalleryList.get(0);
goods.setGoodsThumb(gallery.getThumbUrl());
goods.setGoodsImg(gallery.getImgUrl());
goods.setOriginalImg(gallery.getImgOriginal());
}
Bean2Utils.printEntity(goods);
entity.setGoods(goods);
entity.setGoodsAttrList(goodsAttrList);
entity.setGoodsGalleryList(goodsGalleryList);
JSONObject jsonObject = JSONObject.fromObject(entity);
System.out.println(jsonObject.toString());
Map<String, String> paramsMap = new HashMap<String,String>();
paramsMap.put("json", jsonObject.toString());
String api = request.getScheme()+"://"+ request.getServerName()+":"+request.getServerPort()+"/api/goodsAttr";
// String api = "http://localhost:8087/api/goods";
String apiresult = EHttpClientUtil.httpPost(api, paramsMap);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
//单商品的地址请求
@ResponseBody
@RequestMapping("/getGoodsUrl")
public String getGoodsUrl(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "catId", required = true) Integer catId,
@RequestParam(value = "goodsurl", required = true) String goodsurl
){
try{
return goodsModel(request,goodsurl,catId);
}catch(Exception e){
e.printStackTrace();
}
return "";
}
public static void main(String[] args) throws Exception {
String goodsurl = "https://www.net-a-porter.com/cn/zh/d/Shop/Lingerie/All?cm_sp=topnav-_-clothing-_-lingerie";
NetAPorterController ac = new NetAPorterController();
// ac.goodsModel(url,1);
ac.goodsUrl(null, goodsurl, 1);
}
}
<file_sep>/school-weixin/src/main/java/com/ehais/schoolweixin/controller/verifycodeController.java
package com.ehais.schoolweixin.controller;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class verifycodeController {
@RequestMapping("/getVerifyCode")
public void getCode(HttpServletRequest request,HttpServletResponse response) throws Exception{
//System.out.println("1");
response.setContentType("image/jpeg");
response.setHeader("Pragma", "no-cache");
response.setHeader("Cache-Control","no-cache");
response.setDateHeader("Expires", 0);
//System.out.println("2");
verificationcode vcode=new verificationcode();
vcode.createCode();
HttpSession session = request.getSession();
//System.out.println("3");
session.removeAttribute("validateCode");
vcode.write(response.getOutputStream());
session.setAttribute("validateCode", vcode.getCode());
vcode.write(response.getOutputStream());
//System.out.println("4");
}
@RequestMapping("/hellworld")
@ResponseBody
public String helloworld(){
return "helloworld testing";
}
}
<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/service/HaiArticleService.java
package com.ehais.hrlucene.service;
import org.ehais.tools.ReturnObject;
import com.ehais.hrlucene.model.HaiArticle;
import com.ehais.hrlucene.model.HaiArticleCat;
/**
* @author stephen
*
*/
public interface HaiArticleService {
public ReturnObject<HaiArticle> Save_Article(HaiArticle haiArticle) throws Exception;
public ReturnObject<HaiArticleCat> save_cat(HaiArticleCat haiArticleCat);
}<file_sep>/eh-common/src/main/java/org/ehais/model/ThinkRole.java
package org.ehais.model;
import java.io.Serializable;
import java.util.Date;
public class ThinkRole implements Serializable {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column think_role.id
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
private Short id;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column think_role.name
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
private String name;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column think_role.pid
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
private Short pid;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column think_role.role_code
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
private String roleCode;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column think_role.status
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
private Boolean status;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column think_role.remark
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
private String remark;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column think_role.create_time
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
private Date createTime;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column think_role.update_time
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
private Date updateTime;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table think_role
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
private static final long serialVersionUID = 1L;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column think_role.id
*
* @return the value of think_role.id
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public Short getId() {
return id;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column think_role.id
*
* @param id the value for think_role.id
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public void setId(Short id) {
this.id = id;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column think_role.name
*
* @return the value of think_role.name
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public String getName() {
return name;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column think_role.name
*
* @param name the value for think_role.name
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public void setName(String name) {
this.name = name;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column think_role.pid
*
* @return the value of think_role.pid
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public Short getPid() {
return pid;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column think_role.pid
*
* @param pid the value for think_role.pid
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public void setPid(Short pid) {
this.pid = pid;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column think_role.role_code
*
* @return the value of think_role.role_code
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public String getRoleCode() {
return roleCode;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column think_role.role_code
*
* @param roleCode the value for think_role.role_code
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public void setRoleCode(String roleCode) {
this.roleCode = roleCode;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column think_role.status
*
* @return the value of think_role.status
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public Boolean getStatus() {
return status;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column think_role.status
*
* @param status the value for think_role.status
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public void setStatus(Boolean status) {
this.status = status;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column think_role.remark
*
* @return the value of think_role.remark
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public String getRemark() {
return remark;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column think_role.remark
*
* @param remark the value for think_role.remark
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public void setRemark(String remark) {
this.remark = remark;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column think_role.create_time
*
* @return the value of think_role.create_time
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public Date getCreateTime() {
return createTime;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column think_role.create_time
*
* @param createTime the value for think_role.create_time
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public void setCreateTime(Date createTime) {
this.createTime = createTime;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column think_role.update_time
*
* @return the value of think_role.update_time
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public Date getUpdateTime() {
return updateTime;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column think_role.update_time
*
* @param updateTime the value for think_role.update_time
*
* @mbggenerated Mon Apr 11 23:09:51 CST 2016
*/
public void setUpdateTime(Date updateTime) {
this.updateTime = updateTime;
}
}<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/IndexFiGoController.java
package com.ehais.figoarticle.controller;
import java.net.URLEncoder;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.ehais.controller.CommonController;
import org.ehais.tools.ReturnObject;
import org.ehais.util.DateUtil;
import org.ehais.util.EHtmlUnit;
import org.ehais.util.EHttpClientUtil;
import org.ehais.util.HtmlRegexpUtil;
import org.ehais.util.PythonUtil;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.mapper.ArticleCommentMapper;
import com.ehais.figoarticle.mapper.ArticleMapper;
import com.ehais.figoarticle.mapper.BrandMapper;
import com.ehais.figoarticle.mapper.ExcelMapper;
import com.ehais.figoarticle.model.Article;
import com.ehais.figoarticle.model.ArticleComment;
import com.ehais.figoarticle.model.ArticleExample;
import com.ehais.figoarticle.model.Brand;
import com.ehais.figoarticle.model.Excel;
import com.ehais.figoarticle.model.ExcelExample;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
@Controller
@RequestMapping("/")
public class IndexFiGoController extends CommonController {
@Autowired
private ArticleMapper articleMapper;
@Autowired
private ArticleCommentMapper articleCommentMapper;
@Autowired
private ExcelMapper excelMapper;
@Autowired
private BrandMapper brandMapper;
@RequestMapping("/index.html")
public String index(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response) {
try {
} catch (Exception e) {
e.printStackTrace();
}
return "/index";
}
@ResponseBody
@RequestMapping("/article.api")
public String article(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response,
@RequestParam(value = "page", required = true) Integer page,
@RequestParam(value = "rows", required = true) Integer rows) {
ReturnObject<Article> rm = new ReturnObject<Article>();
try {
ArticleExample example = new ArticleExample();
example.setLimitStart((page - 1) * rows);
example.setLimitEnd(rows);
example.setOrderByClause("created desc");
List<Article> list = articleMapper.selectByExample(example);
Integer total = Long.valueOf(articleMapper.countByExample(example)).intValue();
rm.setTotal(total);
rm.setRows(list);
return this.writeJson(rm);
} catch (Exception e) {
e.printStackTrace();
}
return "";
}
@ResponseBody
@RequestMapping("/toutiaoApiDB")
public String toutiaoApiDB(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response,
@RequestParam(value = "keyword", required = true) String keyword,
@RequestParam(value = "count", required = true) Integer count,
@RequestParam(value = "offset", required = true) Integer offset) {
// response.setHeader("Access-Control-Allow-Origin", "*");
// response.setHeader("Access-Control-Allow-Methods", "POST, GET, OPTIONS, DELETE");
// response.setHeader("Access-Control-Max-Age", "3600");
// response.setHeader("Access-Control-Allow-Headers", "x-requested-with");
response.setHeader("Access-Control-Allow-Origin","*"); // 允许任意域名发起的跨域请求
response.setHeader("Access-Control-Allow-Headers","X-Requested-With,X_Requested_With");
String errobj = null;
try {
String url = "http://www.toutiao.com/search_content/?format=json&keyword="+URLEncoder.encode(keyword, "UTF-8")+"&count="+count+"&offset="+offset;
System.out.println(url);
Map<String, Integer> brandMap = new HashMap<String, Integer>();
List<Brand> brandList = brandMapper.selectByExample(null);
for (Brand b : brandList) {
brandMap.put(b.getName().trim(), b.getId());
}
// String result = EHtmlUnit.getAjaxPage(url);//EHttpClientUtil.methodGet(url);
String result = PythonUtil.python(request.getRealPath("/getAjaxWeb.py"), url);
System.out.println("result=========================");
System.out.println(result);
result = HtmlRegexpUtil.filterHtml(result);
System.out.println(result);
JSONObject json = JSONObject.fromObject(result);
JSONArray arr = json.getJSONArray("data");
System.out.println(keyword+"=="+json.getString("return_count"));
for (Object object : arr) {
errobj = object.toString();
Article a = new Article();
JSONObject o = (JSONObject)object;
if(!o.has("id"))continue;
// System.out.println(o.getString("id"));
a.setWid(o.getLong("id"));
if(o.has("group_id"))a.setGid(o.getLong("group_id"));
if(o.has("item_id"))a.setItemId(o.getLong("item_id"));
if(!o.has("title"))continue;
a.setTitle(o.getString("title"));
a.setSiteId(1L);
if(o.has("abstract"))a.setAbstracts(o.getString("abstract"));
if(o.has("source"))a.setAuthor(o.getString("source"));
a.setReply(o.getInt("comments_count"));
a.setPreReply(0);
a.setSeplyStatus(0);
a.setBrandId(brandMap.get(keyword.trim())==null?0:brandMap.get(keyword.trim()));
a.setKeyword(o.getString("keywords"));
a.setPositive(2);
a.setStatus(0);
if(o.has("url"))a.setUrl(o.getString("url"));
if(o.has("share_url"))a.setShareUrl(o.getString("share_url"));
if(o.has("datetime")){
a.setReleaseTime(Long.valueOf(DateUtil.dateToStamp(o.getString("datetime")+":00") / 1000).intValue());
}else{
a.setReleaseTime(0);
}
a.setCreated(Long.valueOf(System.currentTimeMillis() / 1000).intValue());
a.setUpdated(0);
a.setDeleted(0);
a.setIsDanger(0);
ArticleExample example = new ArticleExample();
example.createCriteria().andWidEqualTo(a.getWid());
long c = articleMapper.countByExample(example);
if(c == 0){
articleMapper.insert(a);
}else{
List<Article> list = articleMapper.selectByExample(example);
Article article = list.get(0);
article.setPreReply(article.getReply());
article.setReply(a.getReply());
articleMapper.updateByPrimaryKey(article);
}
}
return "{\"code\":1,\"message\":\"成功\"}";
// return this.writeJsonObject(json);
} catch (Exception e) {
e.printStackTrace();
System.out.println(errobj);
}
return "{\"code\":2,\"message\":\"失败\"}";
}
@ResponseBody
@RequestMapping("/toutiaoApi")
public String toutiaoApi(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response,
@RequestParam(value = "keyword", required = true) String keyword,
@RequestParam(value = "count", required = true) Integer count,
@RequestParam(value = "offset", required = true) Integer offset) {
// response.setHeader("Access-Control-Allow-Origin", "*");
// response.setHeader("Access-Control-Allow-Methods", "POST, GET, OPTIONS, DELETE");
// response.setHeader("Access-Control-Max-Age", "3600");
// response.setHeader("Access-Control-Allow-Headers", "x-requested-with");
response.setHeader("Access-Control-Allow-Origin","*"); // 允许任意域名发起的跨域请求
response.setHeader("Access-Control-Allow-Headers","X-Requested-With,X_Requested_With");
String url = "http://www.toutiao.com/search_content/?format=json&keyword="+keyword+"&count="+count+"&offset="+offset;
System.out.println(url);
String errobj = null;
try {
String result = EHttpClientUtil.methodGet(url);
return result;
} catch (Exception e) {
e.printStackTrace();
System.out.println(errobj);
}
return "{\"code\":2,\"message\":\"失败\"}";
}
@ResponseBody
@RequestMapping("/baidu")
public String baidu(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response) {
try{
ExcelExample example = new ExcelExample();
example.createCriteria().andValidEqualTo(Integer.valueOf(1).shortValue());
List<Excel> list = excelMapper.selectByExample(example);
String url , result = null;
Integer baidu_count , news_count = 0;
for (Excel excel : list) {
baidu_count = 0 ; news_count = 0;
url = "http://www.baidu.com/s?wd="+java.net.URLEncoder.encode(excel.getUrl(), "UTF-8");
System.out.println(url);
result = EHtmlUnit.httpUnitRequest(url);
Document doc = Jsoup.parse(result);
Element content_left = doc.getElementById("content_left");
Elements container = content_left.getElementsByClass("c-container");
baidu_count = container.size();
url = "http://news.baidu.com/ns?tn=news&word="+java.net.URLEncoder.encode(excel.getUrl(), "UTF-8");
System.out.println(url);
result = EHtmlUnit.httpUnitRequest(url);
// System.out.println(result);
Document docx = Jsoup.parse(result,"UTF-8");
Elements resultx = docx.getElementById("content_left").getElementsByClass("result");
news_count = resultx.size();
ExcelExample examplex = new ExcelExample();
example.createCriteria().andIdEqualTo(excel.getId());
excel.setValid(Integer.valueOf(-1).shortValue());
excel.setBaiduCount(baidu_count);
excel.setNewsCount(news_count);
excelMapper.updateByExample(excel, examplex);
}
}catch(Exception e){
e.printStackTrace();
}
return "{\"code\":1}";
}
@ResponseBody
@RequestMapping("/comment")
public String comment(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "article_id", required = true) Long article_id) {
try {
ArticleExample example = new ArticleExample();
example.createCriteria().andIdEqualTo(article_id);
List<Article> list = articleMapper.selectByExample(example);
for (Article article : list) {
String result = this.commentApi(article.getWid(), article.getItemId(), 0, 200);
System.out.println(result);
JSONObject json = JSONObject.fromObject(result);
JSONObject jsonData = json.getJSONObject("data");
JSONArray comments = jsonData.getJSONArray("comments");
for (Object object : comments) {
JSONObject o = (JSONObject)object;
String text = removeFourChar(o.getString("text"));
ArticleComment comment = new ArticleComment();
comment.setArticleId(article.getId());
comment.setCommentContent(text);
comment.setStatus(1);
comment.setSiteId(1);//头条
comment.setDeleted(0);
comment.setUrl(article.getUrl());
Integer dateint = Long.valueOf(System.currentTimeMillis() / 1000).intValue();
comment.setUpdated(dateint);
comment.setCreated(dateint);
articleCommentMapper.insert(comment);
}
}
return "success";
} catch (Exception e) {
e.printStackTrace();
}
return "{\"code\":2,\"message\":\"失败\"}";
}
@ResponseBody
@RequestMapping("/commentApi")
public String commentApi(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response,
@RequestParam(value = "group_id", required = true) Long group_id,
@RequestParam(value = "item_id", required = true) Long item_id,
@RequestParam(value = "count", required = true) Integer count,
@RequestParam(value = "offset", required = true) Integer offset) {
response.setHeader("Access-Control-Allow-Origin","*"); // 允许任意域名发起的跨域请求
response.setHeader("Access-Control-Allow-Headers","X-Requested-With,X_Requested_With");
String url = "http://www.toutiao.com/api/comment/list/?group_id="+group_id+"&item_id="+item_id+"&count="+count+"&offset="+offset;
String result = null;
String errobj = null;
try {
result = commentApi(group_id,item_id,offset,count);
return result;
} catch (Exception e) {
e.printStackTrace();
System.out.println(errobj);
}
return "{\"code\":2,\"message\":\"失败\"}";
}
private String commentApi(Long group_id,Long item_id,Integer offset,Integer count) throws Exception{
String url = "http://www.toutiao.com/api/comment/list/?group_id="+group_id.toString()+"&item_id="+item_id.toString()+"&count="+count.toString()+"&offset="+offset.toString();
System.out.println(url);
return EHttpClientUtil.methodGet(url);
}
public String removeFourChar(String content) {
byte[] conbyte = content.getBytes();
for (int i = 0; i < conbyte.length; i++) {
if ((conbyte[i] & 0xF8) == 0xF0) {
for (int j = 0; j < 4; j++) {
conbyte[i+j]=0x30;
}
i += 3;
}
}
content = new String(conbyte);
return content.replaceAll("0000", "");
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/model/ArticleExample.java
package com.ehais.figoarticle.model;
import java.util.ArrayList;
import java.util.List;
public class ArticleExample {
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
protected String orderByClause;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
protected boolean distinct;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
protected List<Criteria> oredCriteria;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
protected int limitStart = -1;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
protected int limitEnd = -1;
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public ArticleExample() {
oredCriteria = new ArrayList<Criteria>();
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public String getOrderByClause() {
return orderByClause;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public boolean isDistinct() {
return distinct;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setLimitStart(int limitStart) {
this.limitStart=limitStart;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public int getLimitStart() {
return limitStart;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public void setLimitEnd(int limitEnd) {
this.limitEnd=limitEnd;
}
/**
* This method was generated by MyBatis Generator.
* This method corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public int getLimitEnd() {
return limitEnd;
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andIdIsNull() {
addCriterion("id is null");
return (Criteria) this;
}
public Criteria andIdIsNotNull() {
addCriterion("id is not null");
return (Criteria) this;
}
public Criteria andIdEqualTo(Long value) {
addCriterion("id =", value, "id");
return (Criteria) this;
}
public Criteria andIdNotEqualTo(Long value) {
addCriterion("id <>", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThan(Long value) {
addCriterion("id >", value, "id");
return (Criteria) this;
}
public Criteria andIdGreaterThanOrEqualTo(Long value) {
addCriterion("id >=", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThan(Long value) {
addCriterion("id <", value, "id");
return (Criteria) this;
}
public Criteria andIdLessThanOrEqualTo(Long value) {
addCriterion("id <=", value, "id");
return (Criteria) this;
}
public Criteria andIdIn(List<Long> values) {
addCriterion("id in", values, "id");
return (Criteria) this;
}
public Criteria andIdNotIn(List<Long> values) {
addCriterion("id not in", values, "id");
return (Criteria) this;
}
public Criteria andIdBetween(Long value1, Long value2) {
addCriterion("id between", value1, value2, "id");
return (Criteria) this;
}
public Criteria andIdNotBetween(Long value1, Long value2) {
addCriterion("id not between", value1, value2, "id");
return (Criteria) this;
}
public Criteria andSiteIdIsNull() {
addCriterion("site_id is null");
return (Criteria) this;
}
public Criteria andSiteIdIsNotNull() {
addCriterion("site_id is not null");
return (Criteria) this;
}
public Criteria andSiteIdEqualTo(Long value) {
addCriterion("site_id =", value, "siteId");
return (Criteria) this;
}
public Criteria andSiteIdNotEqualTo(Long value) {
addCriterion("site_id <>", value, "siteId");
return (Criteria) this;
}
public Criteria andSiteIdGreaterThan(Long value) {
addCriterion("site_id >", value, "siteId");
return (Criteria) this;
}
public Criteria andSiteIdGreaterThanOrEqualTo(Long value) {
addCriterion("site_id >=", value, "siteId");
return (Criteria) this;
}
public Criteria andSiteIdLessThan(Long value) {
addCriterion("site_id <", value, "siteId");
return (Criteria) this;
}
public Criteria andSiteIdLessThanOrEqualTo(Long value) {
addCriterion("site_id <=", value, "siteId");
return (Criteria) this;
}
public Criteria andSiteIdIn(List<Long> values) {
addCriterion("site_id in", values, "siteId");
return (Criteria) this;
}
public Criteria andSiteIdNotIn(List<Long> values) {
addCriterion("site_id not in", values, "siteId");
return (Criteria) this;
}
public Criteria andSiteIdBetween(Long value1, Long value2) {
addCriterion("site_id between", value1, value2, "siteId");
return (Criteria) this;
}
public Criteria andSiteIdNotBetween(Long value1, Long value2) {
addCriterion("site_id not between", value1, value2, "siteId");
return (Criteria) this;
}
public Criteria andWidIsNull() {
addCriterion("wid is null");
return (Criteria) this;
}
public Criteria andWidIsNotNull() {
addCriterion("wid is not null");
return (Criteria) this;
}
public Criteria andWidEqualTo(Long value) {
addCriterion("wid =", value, "wid");
return (Criteria) this;
}
public Criteria andWidNotEqualTo(Long value) {
addCriterion("wid <>", value, "wid");
return (Criteria) this;
}
public Criteria andWidGreaterThan(Long value) {
addCriterion("wid >", value, "wid");
return (Criteria) this;
}
public Criteria andWidGreaterThanOrEqualTo(Long value) {
addCriterion("wid >=", value, "wid");
return (Criteria) this;
}
public Criteria andWidLessThan(Long value) {
addCriterion("wid <", value, "wid");
return (Criteria) this;
}
public Criteria andWidLessThanOrEqualTo(Long value) {
addCriterion("wid <=", value, "wid");
return (Criteria) this;
}
public Criteria andWidIn(List<Long> values) {
addCriterion("wid in", values, "wid");
return (Criteria) this;
}
public Criteria andWidNotIn(List<Long> values) {
addCriterion("wid not in", values, "wid");
return (Criteria) this;
}
public Criteria andWidBetween(Long value1, Long value2) {
addCriterion("wid between", value1, value2, "wid");
return (Criteria) this;
}
public Criteria andWidNotBetween(Long value1, Long value2) {
addCriterion("wid not between", value1, value2, "wid");
return (Criteria) this;
}
public Criteria andGidIsNull() {
addCriterion("gid is null");
return (Criteria) this;
}
public Criteria andGidIsNotNull() {
addCriterion("gid is not null");
return (Criteria) this;
}
public Criteria andGidEqualTo(Long value) {
addCriterion("gid =", value, "gid");
return (Criteria) this;
}
public Criteria andGidNotEqualTo(Long value) {
addCriterion("gid <>", value, "gid");
return (Criteria) this;
}
public Criteria andGidGreaterThan(Long value) {
addCriterion("gid >", value, "gid");
return (Criteria) this;
}
public Criteria andGidGreaterThanOrEqualTo(Long value) {
addCriterion("gid >=", value, "gid");
return (Criteria) this;
}
public Criteria andGidLessThan(Long value) {
addCriterion("gid <", value, "gid");
return (Criteria) this;
}
public Criteria andGidLessThanOrEqualTo(Long value) {
addCriterion("gid <=", value, "gid");
return (Criteria) this;
}
public Criteria andGidIn(List<Long> values) {
addCriterion("gid in", values, "gid");
return (Criteria) this;
}
public Criteria andGidNotIn(List<Long> values) {
addCriterion("gid not in", values, "gid");
return (Criteria) this;
}
public Criteria andGidBetween(Long value1, Long value2) {
addCriterion("gid between", value1, value2, "gid");
return (Criteria) this;
}
public Criteria andGidNotBetween(Long value1, Long value2) {
addCriterion("gid not between", value1, value2, "gid");
return (Criteria) this;
}
public Criteria andTitleIsNull() {
addCriterion("title is null");
return (Criteria) this;
}
public Criteria andTitleIsNotNull() {
addCriterion("title is not null");
return (Criteria) this;
}
public Criteria andTitleEqualTo(String value) {
addCriterion("title =", value, "title");
return (Criteria) this;
}
public Criteria andTitleNotEqualTo(String value) {
addCriterion("title <>", value, "title");
return (Criteria) this;
}
public Criteria andTitleGreaterThan(String value) {
addCriterion("title >", value, "title");
return (Criteria) this;
}
public Criteria andTitleGreaterThanOrEqualTo(String value) {
addCriterion("title >=", value, "title");
return (Criteria) this;
}
public Criteria andTitleLessThan(String value) {
addCriterion("title <", value, "title");
return (Criteria) this;
}
public Criteria andTitleLessThanOrEqualTo(String value) {
addCriterion("title <=", value, "title");
return (Criteria) this;
}
public Criteria andTitleLike(String value) {
addCriterion("title like", value, "title");
return (Criteria) this;
}
public Criteria andTitleNotLike(String value) {
addCriterion("title not like", value, "title");
return (Criteria) this;
}
public Criteria andTitleIn(List<String> values) {
addCriterion("title in", values, "title");
return (Criteria) this;
}
public Criteria andTitleNotIn(List<String> values) {
addCriterion("title not in", values, "title");
return (Criteria) this;
}
public Criteria andTitleBetween(String value1, String value2) {
addCriterion("title between", value1, value2, "title");
return (Criteria) this;
}
public Criteria andTitleNotBetween(String value1, String value2) {
addCriterion("title not between", value1, value2, "title");
return (Criteria) this;
}
public Criteria andAuthorIsNull() {
addCriterion("author is null");
return (Criteria) this;
}
public Criteria andAuthorIsNotNull() {
addCriterion("author is not null");
return (Criteria) this;
}
public Criteria andAuthorEqualTo(String value) {
addCriterion("author =", value, "author");
return (Criteria) this;
}
public Criteria andAuthorNotEqualTo(String value) {
addCriterion("author <>", value, "author");
return (Criteria) this;
}
public Criteria andAuthorGreaterThan(String value) {
addCriterion("author >", value, "author");
return (Criteria) this;
}
public Criteria andAuthorGreaterThanOrEqualTo(String value) {
addCriterion("author >=", value, "author");
return (Criteria) this;
}
public Criteria andAuthorLessThan(String value) {
addCriterion("author <", value, "author");
return (Criteria) this;
}
public Criteria andAuthorLessThanOrEqualTo(String value) {
addCriterion("author <=", value, "author");
return (Criteria) this;
}
public Criteria andAuthorLike(String value) {
addCriterion("author like", value, "author");
return (Criteria) this;
}
public Criteria andAuthorNotLike(String value) {
addCriterion("author not like", value, "author");
return (Criteria) this;
}
public Criteria andAuthorIn(List<String> values) {
addCriterion("author in", values, "author");
return (Criteria) this;
}
public Criteria andAuthorNotIn(List<String> values) {
addCriterion("author not in", values, "author");
return (Criteria) this;
}
public Criteria andAuthorBetween(String value1, String value2) {
addCriterion("author between", value1, value2, "author");
return (Criteria) this;
}
public Criteria andAuthorNotBetween(String value1, String value2) {
addCriterion("author not between", value1, value2, "author");
return (Criteria) this;
}
public Criteria andReplyIsNull() {
addCriterion("reply is null");
return (Criteria) this;
}
public Criteria andReplyIsNotNull() {
addCriterion("reply is not null");
return (Criteria) this;
}
public Criteria andReplyEqualTo(Integer value) {
addCriterion("reply =", value, "reply");
return (Criteria) this;
}
public Criteria andReplyNotEqualTo(Integer value) {
addCriterion("reply <>", value, "reply");
return (Criteria) this;
}
public Criteria andReplyGreaterThan(Integer value) {
addCriterion("reply >", value, "reply");
return (Criteria) this;
}
public Criteria andReplyGreaterThanOrEqualTo(Integer value) {
addCriterion("reply >=", value, "reply");
return (Criteria) this;
}
public Criteria andReplyLessThan(Integer value) {
addCriterion("reply <", value, "reply");
return (Criteria) this;
}
public Criteria andReplyLessThanOrEqualTo(Integer value) {
addCriterion("reply <=", value, "reply");
return (Criteria) this;
}
public Criteria andReplyIn(List<Integer> values) {
addCriterion("reply in", values, "reply");
return (Criteria) this;
}
public Criteria andReplyNotIn(List<Integer> values) {
addCriterion("reply not in", values, "reply");
return (Criteria) this;
}
public Criteria andReplyBetween(Integer value1, Integer value2) {
addCriterion("reply between", value1, value2, "reply");
return (Criteria) this;
}
public Criteria andReplyNotBetween(Integer value1, Integer value2) {
addCriterion("reply not between", value1, value2, "reply");
return (Criteria) this;
}
public Criteria andPreReplyIsNull() {
addCriterion("pre_reply is null");
return (Criteria) this;
}
public Criteria andPreReplyIsNotNull() {
addCriterion("pre_reply is not null");
return (Criteria) this;
}
public Criteria andPreReplyEqualTo(Integer value) {
addCriterion("pre_reply =", value, "preReply");
return (Criteria) this;
}
public Criteria andPreReplyNotEqualTo(Integer value) {
addCriterion("pre_reply <>", value, "preReply");
return (Criteria) this;
}
public Criteria andPreReplyGreaterThan(Integer value) {
addCriterion("pre_reply >", value, "preReply");
return (Criteria) this;
}
public Criteria andPreReplyGreaterThanOrEqualTo(Integer value) {
addCriterion("pre_reply >=", value, "preReply");
return (Criteria) this;
}
public Criteria andPreReplyLessThan(Integer value) {
addCriterion("pre_reply <", value, "preReply");
return (Criteria) this;
}
public Criteria andPreReplyLessThanOrEqualTo(Integer value) {
addCriterion("pre_reply <=", value, "preReply");
return (Criteria) this;
}
public Criteria andPreReplyIn(List<Integer> values) {
addCriterion("pre_reply in", values, "preReply");
return (Criteria) this;
}
public Criteria andPreReplyNotIn(List<Integer> values) {
addCriterion("pre_reply not in", values, "preReply");
return (Criteria) this;
}
public Criteria andPreReplyBetween(Integer value1, Integer value2) {
addCriterion("pre_reply between", value1, value2, "preReply");
return (Criteria) this;
}
public Criteria andPreReplyNotBetween(Integer value1, Integer value2) {
addCriterion("pre_reply not between", value1, value2, "preReply");
return (Criteria) this;
}
public Criteria andSeplyStatusIsNull() {
addCriterion("seply_status is null");
return (Criteria) this;
}
public Criteria andSeplyStatusIsNotNull() {
addCriterion("seply_status is not null");
return (Criteria) this;
}
public Criteria andSeplyStatusEqualTo(Integer value) {
addCriterion("seply_status =", value, "seplyStatus");
return (Criteria) this;
}
public Criteria andSeplyStatusNotEqualTo(Integer value) {
addCriterion("seply_status <>", value, "seplyStatus");
return (Criteria) this;
}
public Criteria andSeplyStatusGreaterThan(Integer value) {
addCriterion("seply_status >", value, "seplyStatus");
return (Criteria) this;
}
public Criteria andSeplyStatusGreaterThanOrEqualTo(Integer value) {
addCriterion("seply_status >=", value, "seplyStatus");
return (Criteria) this;
}
public Criteria andSeplyStatusLessThan(Integer value) {
addCriterion("seply_status <", value, "seplyStatus");
return (Criteria) this;
}
public Criteria andSeplyStatusLessThanOrEqualTo(Integer value) {
addCriterion("seply_status <=", value, "seplyStatus");
return (Criteria) this;
}
public Criteria andSeplyStatusIn(List<Integer> values) {
addCriterion("seply_status in", values, "seplyStatus");
return (Criteria) this;
}
public Criteria andSeplyStatusNotIn(List<Integer> values) {
addCriterion("seply_status not in", values, "seplyStatus");
return (Criteria) this;
}
public Criteria andSeplyStatusBetween(Integer value1, Integer value2) {
addCriterion("seply_status between", value1, value2, "seplyStatus");
return (Criteria) this;
}
public Criteria andSeplyStatusNotBetween(Integer value1, Integer value2) {
addCriterion("seply_status not between", value1, value2, "seplyStatus");
return (Criteria) this;
}
public Criteria andBrandIdIsNull() {
addCriterion("brand_id is null");
return (Criteria) this;
}
public Criteria andBrandIdIsNotNull() {
addCriterion("brand_id is not null");
return (Criteria) this;
}
public Criteria andBrandIdEqualTo(Integer value) {
addCriterion("brand_id =", value, "brandId");
return (Criteria) this;
}
public Criteria andBrandIdNotEqualTo(Integer value) {
addCriterion("brand_id <>", value, "brandId");
return (Criteria) this;
}
public Criteria andBrandIdGreaterThan(Integer value) {
addCriterion("brand_id >", value, "brandId");
return (Criteria) this;
}
public Criteria andBrandIdGreaterThanOrEqualTo(Integer value) {
addCriterion("brand_id >=", value, "brandId");
return (Criteria) this;
}
public Criteria andBrandIdLessThan(Integer value) {
addCriterion("brand_id <", value, "brandId");
return (Criteria) this;
}
public Criteria andBrandIdLessThanOrEqualTo(Integer value) {
addCriterion("brand_id <=", value, "brandId");
return (Criteria) this;
}
public Criteria andBrandIdIn(List<Integer> values) {
addCriterion("brand_id in", values, "brandId");
return (Criteria) this;
}
public Criteria andBrandIdNotIn(List<Integer> values) {
addCriterion("brand_id not in", values, "brandId");
return (Criteria) this;
}
public Criteria andBrandIdBetween(Integer value1, Integer value2) {
addCriterion("brand_id between", value1, value2, "brandId");
return (Criteria) this;
}
public Criteria andBrandIdNotBetween(Integer value1, Integer value2) {
addCriterion("brand_id not between", value1, value2, "brandId");
return (Criteria) this;
}
public Criteria andPositiveIsNull() {
addCriterion("positive is null");
return (Criteria) this;
}
public Criteria andPositiveIsNotNull() {
addCriterion("positive is not null");
return (Criteria) this;
}
public Criteria andPositiveEqualTo(Integer value) {
addCriterion("positive =", value, "positive");
return (Criteria) this;
}
public Criteria andPositiveNotEqualTo(Integer value) {
addCriterion("positive <>", value, "positive");
return (Criteria) this;
}
public Criteria andPositiveGreaterThan(Integer value) {
addCriterion("positive >", value, "positive");
return (Criteria) this;
}
public Criteria andPositiveGreaterThanOrEqualTo(Integer value) {
addCriterion("positive >=", value, "positive");
return (Criteria) this;
}
public Criteria andPositiveLessThan(Integer value) {
addCriterion("positive <", value, "positive");
return (Criteria) this;
}
public Criteria andPositiveLessThanOrEqualTo(Integer value) {
addCriterion("positive <=", value, "positive");
return (Criteria) this;
}
public Criteria andPositiveIn(List<Integer> values) {
addCriterion("positive in", values, "positive");
return (Criteria) this;
}
public Criteria andPositiveNotIn(List<Integer> values) {
addCriterion("positive not in", values, "positive");
return (Criteria) this;
}
public Criteria andPositiveBetween(Integer value1, Integer value2) {
addCriterion("positive between", value1, value2, "positive");
return (Criteria) this;
}
public Criteria andPositiveNotBetween(Integer value1, Integer value2) {
addCriterion("positive not between", value1, value2, "positive");
return (Criteria) this;
}
public Criteria andStatusIsNull() {
addCriterion("status is null");
return (Criteria) this;
}
public Criteria andStatusIsNotNull() {
addCriterion("status is not null");
return (Criteria) this;
}
public Criteria andStatusEqualTo(Integer value) {
addCriterion("status =", value, "status");
return (Criteria) this;
}
public Criteria andStatusNotEqualTo(Integer value) {
addCriterion("status <>", value, "status");
return (Criteria) this;
}
public Criteria andStatusGreaterThan(Integer value) {
addCriterion("status >", value, "status");
return (Criteria) this;
}
public Criteria andStatusGreaterThanOrEqualTo(Integer value) {
addCriterion("status >=", value, "status");
return (Criteria) this;
}
public Criteria andStatusLessThan(Integer value) {
addCriterion("status <", value, "status");
return (Criteria) this;
}
public Criteria andStatusLessThanOrEqualTo(Integer value) {
addCriterion("status <=", value, "status");
return (Criteria) this;
}
public Criteria andStatusIn(List<Integer> values) {
addCriterion("status in", values, "status");
return (Criteria) this;
}
public Criteria andStatusNotIn(List<Integer> values) {
addCriterion("status not in", values, "status");
return (Criteria) this;
}
public Criteria andStatusBetween(Integer value1, Integer value2) {
addCriterion("status between", value1, value2, "status");
return (Criteria) this;
}
public Criteria andStatusNotBetween(Integer value1, Integer value2) {
addCriterion("status not between", value1, value2, "status");
return (Criteria) this;
}
public Criteria andUrlIsNull() {
addCriterion("url is null");
return (Criteria) this;
}
public Criteria andUrlIsNotNull() {
addCriterion("url is not null");
return (Criteria) this;
}
public Criteria andUrlEqualTo(String value) {
addCriterion("url =", value, "url");
return (Criteria) this;
}
public Criteria andUrlNotEqualTo(String value) {
addCriterion("url <>", value, "url");
return (Criteria) this;
}
public Criteria andUrlGreaterThan(String value) {
addCriterion("url >", value, "url");
return (Criteria) this;
}
public Criteria andUrlGreaterThanOrEqualTo(String value) {
addCriterion("url >=", value, "url");
return (Criteria) this;
}
public Criteria andUrlLessThan(String value) {
addCriterion("url <", value, "url");
return (Criteria) this;
}
public Criteria andUrlLessThanOrEqualTo(String value) {
addCriterion("url <=", value, "url");
return (Criteria) this;
}
public Criteria andUrlLike(String value) {
addCriterion("url like", value, "url");
return (Criteria) this;
}
public Criteria andUrlNotLike(String value) {
addCriterion("url not like", value, "url");
return (Criteria) this;
}
public Criteria andUrlIn(List<String> values) {
addCriterion("url in", values, "url");
return (Criteria) this;
}
public Criteria andUrlNotIn(List<String> values) {
addCriterion("url not in", values, "url");
return (Criteria) this;
}
public Criteria andUrlBetween(String value1, String value2) {
addCriterion("url between", value1, value2, "url");
return (Criteria) this;
}
public Criteria andUrlNotBetween(String value1, String value2) {
addCriterion("url not between", value1, value2, "url");
return (Criteria) this;
}
public Criteria andShareUrlIsNull() {
addCriterion("share_url is null");
return (Criteria) this;
}
public Criteria andShareUrlIsNotNull() {
addCriterion("share_url is not null");
return (Criteria) this;
}
public Criteria andShareUrlEqualTo(String value) {
addCriterion("share_url =", value, "shareUrl");
return (Criteria) this;
}
public Criteria andShareUrlNotEqualTo(String value) {
addCriterion("share_url <>", value, "shareUrl");
return (Criteria) this;
}
public Criteria andShareUrlGreaterThan(String value) {
addCriterion("share_url >", value, "shareUrl");
return (Criteria) this;
}
public Criteria andShareUrlGreaterThanOrEqualTo(String value) {
addCriterion("share_url >=", value, "shareUrl");
return (Criteria) this;
}
public Criteria andShareUrlLessThan(String value) {
addCriterion("share_url <", value, "shareUrl");
return (Criteria) this;
}
public Criteria andShareUrlLessThanOrEqualTo(String value) {
addCriterion("share_url <=", value, "shareUrl");
return (Criteria) this;
}
public Criteria andShareUrlLike(String value) {
addCriterion("share_url like", value, "shareUrl");
return (Criteria) this;
}
public Criteria andShareUrlNotLike(String value) {
addCriterion("share_url not like", value, "shareUrl");
return (Criteria) this;
}
public Criteria andShareUrlIn(List<String> values) {
addCriterion("share_url in", values, "shareUrl");
return (Criteria) this;
}
public Criteria andShareUrlNotIn(List<String> values) {
addCriterion("share_url not in", values, "shareUrl");
return (Criteria) this;
}
public Criteria andShareUrlBetween(String value1, String value2) {
addCriterion("share_url between", value1, value2, "shareUrl");
return (Criteria) this;
}
public Criteria andShareUrlNotBetween(String value1, String value2) {
addCriterion("share_url not between", value1, value2, "shareUrl");
return (Criteria) this;
}
public Criteria andReleaseTimeIsNull() {
addCriterion("release_time is null");
return (Criteria) this;
}
public Criteria andReleaseTimeIsNotNull() {
addCriterion("release_time is not null");
return (Criteria) this;
}
public Criteria andReleaseTimeEqualTo(Integer value) {
addCriterion("release_time =", value, "releaseTime");
return (Criteria) this;
}
public Criteria andReleaseTimeNotEqualTo(Integer value) {
addCriterion("release_time <>", value, "releaseTime");
return (Criteria) this;
}
public Criteria andReleaseTimeGreaterThan(Integer value) {
addCriterion("release_time >", value, "releaseTime");
return (Criteria) this;
}
public Criteria andReleaseTimeGreaterThanOrEqualTo(Integer value) {
addCriterion("release_time >=", value, "releaseTime");
return (Criteria) this;
}
public Criteria andReleaseTimeLessThan(Integer value) {
addCriterion("release_time <", value, "releaseTime");
return (Criteria) this;
}
public Criteria andReleaseTimeLessThanOrEqualTo(Integer value) {
addCriterion("release_time <=", value, "releaseTime");
return (Criteria) this;
}
public Criteria andReleaseTimeIn(List<Integer> values) {
addCriterion("release_time in", values, "releaseTime");
return (Criteria) this;
}
public Criteria andReleaseTimeNotIn(List<Integer> values) {
addCriterion("release_time not in", values, "releaseTime");
return (Criteria) this;
}
public Criteria andReleaseTimeBetween(Integer value1, Integer value2) {
addCriterion("release_time between", value1, value2, "releaseTime");
return (Criteria) this;
}
public Criteria andReleaseTimeNotBetween(Integer value1, Integer value2) {
addCriterion("release_time not between", value1, value2, "releaseTime");
return (Criteria) this;
}
public Criteria andCreatedIsNull() {
addCriterion("created is null");
return (Criteria) this;
}
public Criteria andCreatedIsNotNull() {
addCriterion("created is not null");
return (Criteria) this;
}
public Criteria andCreatedEqualTo(Integer value) {
addCriterion("created =", value, "created");
return (Criteria) this;
}
public Criteria andCreatedNotEqualTo(Integer value) {
addCriterion("created <>", value, "created");
return (Criteria) this;
}
public Criteria andCreatedGreaterThan(Integer value) {
addCriterion("created >", value, "created");
return (Criteria) this;
}
public Criteria andCreatedGreaterThanOrEqualTo(Integer value) {
addCriterion("created >=", value, "created");
return (Criteria) this;
}
public Criteria andCreatedLessThan(Integer value) {
addCriterion("created <", value, "created");
return (Criteria) this;
}
public Criteria andCreatedLessThanOrEqualTo(Integer value) {
addCriterion("created <=", value, "created");
return (Criteria) this;
}
public Criteria andCreatedIn(List<Integer> values) {
addCriterion("created in", values, "created");
return (Criteria) this;
}
public Criteria andCreatedNotIn(List<Integer> values) {
addCriterion("created not in", values, "created");
return (Criteria) this;
}
public Criteria andCreatedBetween(Integer value1, Integer value2) {
addCriterion("created between", value1, value2, "created");
return (Criteria) this;
}
public Criteria andCreatedNotBetween(Integer value1, Integer value2) {
addCriterion("created not between", value1, value2, "created");
return (Criteria) this;
}
public Criteria andUpdatedIsNull() {
addCriterion("updated is null");
return (Criteria) this;
}
public Criteria andUpdatedIsNotNull() {
addCriterion("updated is not null");
return (Criteria) this;
}
public Criteria andUpdatedEqualTo(Integer value) {
addCriterion("updated =", value, "updated");
return (Criteria) this;
}
public Criteria andUpdatedNotEqualTo(Integer value) {
addCriterion("updated <>", value, "updated");
return (Criteria) this;
}
public Criteria andUpdatedGreaterThan(Integer value) {
addCriterion("updated >", value, "updated");
return (Criteria) this;
}
public Criteria andUpdatedGreaterThanOrEqualTo(Integer value) {
addCriterion("updated >=", value, "updated");
return (Criteria) this;
}
public Criteria andUpdatedLessThan(Integer value) {
addCriterion("updated <", value, "updated");
return (Criteria) this;
}
public Criteria andUpdatedLessThanOrEqualTo(Integer value) {
addCriterion("updated <=", value, "updated");
return (Criteria) this;
}
public Criteria andUpdatedIn(List<Integer> values) {
addCriterion("updated in", values, "updated");
return (Criteria) this;
}
public Criteria andUpdatedNotIn(List<Integer> values) {
addCriterion("updated not in", values, "updated");
return (Criteria) this;
}
public Criteria andUpdatedBetween(Integer value1, Integer value2) {
addCriterion("updated between", value1, value2, "updated");
return (Criteria) this;
}
public Criteria andUpdatedNotBetween(Integer value1, Integer value2) {
addCriterion("updated not between", value1, value2, "updated");
return (Criteria) this;
}
public Criteria andDeletedIsNull() {
addCriterion("deleted is null");
return (Criteria) this;
}
public Criteria andDeletedIsNotNull() {
addCriterion("deleted is not null");
return (Criteria) this;
}
public Criteria andDeletedEqualTo(Integer value) {
addCriterion("deleted =", value, "deleted");
return (Criteria) this;
}
public Criteria andDeletedNotEqualTo(Integer value) {
addCriterion("deleted <>", value, "deleted");
return (Criteria) this;
}
public Criteria andDeletedGreaterThan(Integer value) {
addCriterion("deleted >", value, "deleted");
return (Criteria) this;
}
public Criteria andDeletedGreaterThanOrEqualTo(Integer value) {
addCriterion("deleted >=", value, "deleted");
return (Criteria) this;
}
public Criteria andDeletedLessThan(Integer value) {
addCriterion("deleted <", value, "deleted");
return (Criteria) this;
}
public Criteria andDeletedLessThanOrEqualTo(Integer value) {
addCriterion("deleted <=", value, "deleted");
return (Criteria) this;
}
public Criteria andDeletedIn(List<Integer> values) {
addCriterion("deleted in", values, "deleted");
return (Criteria) this;
}
public Criteria andDeletedNotIn(List<Integer> values) {
addCriterion("deleted not in", values, "deleted");
return (Criteria) this;
}
public Criteria andDeletedBetween(Integer value1, Integer value2) {
addCriterion("deleted between", value1, value2, "deleted");
return (Criteria) this;
}
public Criteria andDeletedNotBetween(Integer value1, Integer value2) {
addCriterion("deleted not between", value1, value2, "deleted");
return (Criteria) this;
}
public Criteria andKeywordIsNull() {
addCriterion("keyword is null");
return (Criteria) this;
}
public Criteria andKeywordIsNotNull() {
addCriterion("keyword is not null");
return (Criteria) this;
}
public Criteria andKeywordEqualTo(String value) {
addCriterion("keyword =", value, "keyword");
return (Criteria) this;
}
public Criteria andKeywordNotEqualTo(String value) {
addCriterion("keyword <>", value, "keyword");
return (Criteria) this;
}
public Criteria andKeywordGreaterThan(String value) {
addCriterion("keyword >", value, "keyword");
return (Criteria) this;
}
public Criteria andKeywordGreaterThanOrEqualTo(String value) {
addCriterion("keyword >=", value, "keyword");
return (Criteria) this;
}
public Criteria andKeywordLessThan(String value) {
addCriterion("keyword <", value, "keyword");
return (Criteria) this;
}
public Criteria andKeywordLessThanOrEqualTo(String value) {
addCriterion("keyword <=", value, "keyword");
return (Criteria) this;
}
public Criteria andKeywordLike(String value) {
addCriterion("keyword like", value, "keyword");
return (Criteria) this;
}
public Criteria andKeywordNotLike(String value) {
addCriterion("keyword not like", value, "keyword");
return (Criteria) this;
}
public Criteria andKeywordIn(List<String> values) {
addCriterion("keyword in", values, "keyword");
return (Criteria) this;
}
public Criteria andKeywordNotIn(List<String> values) {
addCriterion("keyword not in", values, "keyword");
return (Criteria) this;
}
public Criteria andKeywordBetween(String value1, String value2) {
addCriterion("keyword between", value1, value2, "keyword");
return (Criteria) this;
}
public Criteria andKeywordNotBetween(String value1, String value2) {
addCriterion("keyword not between", value1, value2, "keyword");
return (Criteria) this;
}
public Criteria andIsDangerIsNull() {
addCriterion("is_danger is null");
return (Criteria) this;
}
public Criteria andIsDangerIsNotNull() {
addCriterion("is_danger is not null");
return (Criteria) this;
}
public Criteria andIsDangerEqualTo(Integer value) {
addCriterion("is_danger =", value, "isDanger");
return (Criteria) this;
}
public Criteria andIsDangerNotEqualTo(Integer value) {
addCriterion("is_danger <>", value, "isDanger");
return (Criteria) this;
}
public Criteria andIsDangerGreaterThan(Integer value) {
addCriterion("is_danger >", value, "isDanger");
return (Criteria) this;
}
public Criteria andIsDangerGreaterThanOrEqualTo(Integer value) {
addCriterion("is_danger >=", value, "isDanger");
return (Criteria) this;
}
public Criteria andIsDangerLessThan(Integer value) {
addCriterion("is_danger <", value, "isDanger");
return (Criteria) this;
}
public Criteria andIsDangerLessThanOrEqualTo(Integer value) {
addCriterion("is_danger <=", value, "isDanger");
return (Criteria) this;
}
public Criteria andIsDangerIn(List<Integer> values) {
addCriterion("is_danger in", values, "isDanger");
return (Criteria) this;
}
public Criteria andIsDangerNotIn(List<Integer> values) {
addCriterion("is_danger not in", values, "isDanger");
return (Criteria) this;
}
public Criteria andIsDangerBetween(Integer value1, Integer value2) {
addCriterion("is_danger between", value1, value2, "isDanger");
return (Criteria) this;
}
public Criteria andIsDangerNotBetween(Integer value1, Integer value2) {
addCriterion("is_danger not between", value1, value2, "isDanger");
return (Criteria) this;
}
public Criteria andItemIdIsNull() {
addCriterion("item_id is null");
return (Criteria) this;
}
public Criteria andItemIdIsNotNull() {
addCriterion("item_id is not null");
return (Criteria) this;
}
public Criteria andItemIdEqualTo(Long value) {
addCriterion("item_id =", value, "itemId");
return (Criteria) this;
}
public Criteria andItemIdNotEqualTo(Long value) {
addCriterion("item_id <>", value, "itemId");
return (Criteria) this;
}
public Criteria andItemIdGreaterThan(Long value) {
addCriterion("item_id >", value, "itemId");
return (Criteria) this;
}
public Criteria andItemIdGreaterThanOrEqualTo(Long value) {
addCriterion("item_id >=", value, "itemId");
return (Criteria) this;
}
public Criteria andItemIdLessThan(Long value) {
addCriterion("item_id <", value, "itemId");
return (Criteria) this;
}
public Criteria andItemIdLessThanOrEqualTo(Long value) {
addCriterion("item_id <=", value, "itemId");
return (Criteria) this;
}
public Criteria andItemIdIn(List<Long> values) {
addCriterion("item_id in", values, "itemId");
return (Criteria) this;
}
public Criteria andItemIdNotIn(List<Long> values) {
addCriterion("item_id not in", values, "itemId");
return (Criteria) this;
}
public Criteria andItemIdBetween(Long value1, Long value2) {
addCriterion("item_id between", value1, value2, "itemId");
return (Criteria) this;
}
public Criteria andItemIdNotBetween(Long value1, Long value2) {
addCriterion("item_id not between", value1, value2, "itemId");
return (Criteria) this;
}
public Criteria andTitleLikeInsensitive(String value) {
addCriterion("upper(title) like", value.toUpperCase(), "title");
return (Criteria) this;
}
public Criteria andAuthorLikeInsensitive(String value) {
addCriterion("upper(author) like", value.toUpperCase(), "author");
return (Criteria) this;
}
public Criteria andUrlLikeInsensitive(String value) {
addCriterion("upper(url) like", value.toUpperCase(), "url");
return (Criteria) this;
}
public Criteria andShareUrlLikeInsensitive(String value) {
addCriterion("upper(share_url) like", value.toUpperCase(), "shareUrl");
return (Criteria) this;
}
public Criteria andKeywordLikeInsensitive(String value) {
addCriterion("upper(keyword) like", value.toUpperCase(), "keyword");
return (Criteria) this;
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table article
*
* @mbg.generated do_not_delete_during_merge Fri Jun 09 22:57:18 CST 2017
*/
public static class Criteria extends GeneratedCriteria {
protected Criteria() {
super();
}
}
/**
* This class was generated by MyBatis Generator.
* This class corresponds to the database table article
*
* @mbg.generated Fri Jun 09 22:57:18 CST 2017
*/
public static class Criterion {
private String condition;
private Object value;
private Object secondValue;
private boolean noValue;
private boolean singleValue;
private boolean betweenValue;
private boolean listValue;
private String typeHandler;
public String getCondition() {
return condition;
}
public Object getValue() {
return value;
}
public Object getSecondValue() {
return secondValue;
}
public boolean isNoValue() {
return noValue;
}
public boolean isSingleValue() {
return singleValue;
}
public boolean isBetweenValue() {
return betweenValue;
}
public boolean isListValue() {
return listValue;
}
public String getTypeHandler() {
return typeHandler;
}
protected Criterion(String condition) {
super();
this.condition = condition;
this.typeHandler = null;
this.noValue = true;
}
protected Criterion(String condition, Object value, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.typeHandler = typeHandler;
if (value instanceof List<?>) {
this.listValue = true;
} else {
this.singleValue = true;
}
}
protected Criterion(String condition, Object value) {
this(condition, value, null);
}
protected Criterion(String condition, Object value, Object secondValue, String typeHandler) {
super();
this.condition = condition;
this.value = value;
this.secondValue = secondValue;
this.typeHandler = typeHandler;
this.betweenValue = true;
}
protected Criterion(String condition, Object value, Object secondValue) {
this(condition, value, secondValue, null);
}
}
}<file_sep>/eHR-Lucene/src/main/java/com/ehais/hrlucene/service/impl/GzrcServiceImpl.java
package com.ehais.hrlucene.service.impl;
import java.util.ArrayList;
import org.ehais.tools.ReturnObject;
import org.ehais.util.EHtmlUnit;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.ehais.hrlucene.model.HaiHrPosition;
import com.ehais.hrlucene.model.HaiHrPositionWithBLOBs;
import com.ehais.hrlucene.service.GzrcService;
import com.ehais.hrlucene.service.HrPositionService;
import net.sf.json.JSONObject;
@Service("GzrcService")
public class GzrcServiceImpl implements GzrcService {
@Autowired
private HrPositionService hrPositionService;
private String website = "http://www.gzrc.gov.cn/";
/* (non-Javadoc)
* @see com.ehais.hrlucene.service.impl.GzrcService#loadGzrc()
*/
public void loadGzrc() throws Exception {
// TODO Auto-generated method stub
String htmlContent = EHtmlUnit.httpUnitRequest(this.website+"SearchResult.php");
//将xml的字符串转化成html可读对象
Document doc = Jsoup.parse(htmlContent,"utf-8");
//根据html的结构读取信息
Element Ele = doc.getElementById("hoverZW");
Elements Eletr = Ele.getElementsByTag("tr");
int first =1;
int line = 0;
for (Element subtr : Eletr) {
if (first == 1){
first = 0;
continue;
}
line = line + 1;
System.out.println(line);
Elements Eletd = subtr.getElementsByTag("td");
//性别
String sex = "1";
try{
sex = Eletd.get(5).text();
}catch(Exception e){
break;
}
Elements subtd = Eletd.get(1).getElementsByTag("a");
String href = website + subtd.attr("href");
positionList(href,sex);
// for(Element subtd : Eletd){
// Elements Elea = subtd.getElementsByTag("a");
// for(Element suba : Elea){
// String href = website + Elea.attr("href");
// positionList(href);
// }
//
// }
}
Elements Page = doc.getElementsByClass("page_style");
Elements subpage = Page.get(0).getElementsByTag("a");
String nextpage = website + subpage.get(11).attr("href");
loadGzrc(nextpage);
}
public void positionList(String urlhr,String a) throws Exception{
System.out.println("请求:" + urlhr);
String htmlContent = EHtmlUnit.httpUnitRequest(urlhr);
Document doc = Jsoup.parse(htmlContent,"utf-8");
Elements poslist = doc.getElementsByClass("tit-h2");
String positionName = poslist.get(0).text();
Elements subpos = doc.getElementsByClass("top-content");
Elements posdl1 = subpos.get(0).getElementsByTag("p");
String profession = posdl1.get(0).text();
profession = profession.replace("专业要求:", "");
String degreelevel = posdl1.get(1).text();
degreelevel = degreelevel.replace("学历要求:", "");
String workyears = posdl1.get(2).text();
workyears = workyears.replace("工作经验:", "");
String salary = posdl1.get(3).text();
salary = salary.replace("薪资待遇:", "");
String headcount = posdl1.get(4).text();
headcount = headcount.replace("招聘人数:", "");
Elements posdl2 = subpos.get(1).getElementsByTag("p");
String issueTime = posdl2.get(0).text();
issueTime = issueTime.replace("更新日期:", "");
String workages = posdl2.get(2).text();
workages = workages.replace("年龄要求:","");
String language = posdl2.get(3).text();
language = language.replace("外语要求:", "");
String workcity = posdl2.get(5).text();
workcity = workcity.replace("工作地点:", "");
Elements PosIntro = doc.getElementsByClass("sesn-info");
String workIntro = PosIntro.get(0).text();
Elements CpyIntro = doc.getElementsByClass("job-company");
String companyDetail = CpyIntro.get(0).text();
Elements Cpyname = doc.getElementsByClass("comp-tit");
String companyName = Cpyname.get(0).text();
Elements Cpyscale = doc.getElementsByClass("s-comp-info");
ArrayList<String> comp = new ArrayList<String>();
for(Element subcpy : Cpyscale){
Elements compscale = subcpy.getElementsByTag("span");
if(compscale.size()==0){
continue;
}
comp.add(compscale.get(0).text());
// System.out.println("stop");
}
String companyScale = "-";
try{
companyScale = comp.get(0);
}catch(Exception e){
}
String companyNature = "-";
try{
companyNature = comp.get(1);
}catch(Exception e){
}
String companyIndustry = "-";
try{
companyIndustry = comp.get(2);
}catch(Exception e){
}
String gender = a;
HaiHrPositionWithBLOBs position = new HaiHrPositionWithBLOBs();
position.setPositionName(positionName);
position.setHeadcount(headcount);
position.setDegreelevel(degreelevel);
position.setSalary(salary);
position.setWorkyears(workyears);
position.setWorkcity(workcity);
position.setProfession(profession);
position.setWorkages(workages);
position.setGender(gender);
position.setLanguage(language);
position.setIssueTime(issueTime);
position.setCompanyName(companyName);
position.setCompanyNature(companyNature);
position.setCompanyScale(companyScale);
position.setCompanyIndustry(companyIndustry);
position.setCompanyDetail(companyDetail);
position.setWorkIntro(workIntro);
position.setHrSource("贵州人才网");
position.setHrPositionUrl(urlhr);
ReturnObject<HaiHrPosition> rm = this.hrPositionService.SaveHrPosition(position);
JSONObject obj = JSONObject.fromObject(rm);
System.out.println(obj.toString());
}
public void loadGzrc(String URLs) throws Exception{
// TODO Auto-generated method stub
String htmlContent = EHtmlUnit.httpUnitRequest(URLs);
//将xml的字符串转化成html可读对象
Document doc = Jsoup.parse(htmlContent,"utf-8");
//根据html的结构读取信息
Element Ele = doc.getElementById("hoverZW");
Elements Eletr = Ele.getElementsByTag("tr");
int first =1;
int line = 0;
for (Element subtr : Eletr) {
if (first == 1){
first=0;
continue;
}
line = line + 1;
System.out.println(line);
Elements Eletd = subtr.getElementsByTag("td");
//性别
String sex = Eletd.get(5).text();
Elements subtd = Eletd.get(1).getElementsByTag("a");
String href = website + subtd.attr("href");
positionList(href,sex);
}
Elements Page = doc.getElementsByClass("page_style mLink");
Elements subpage = Page.get(0).getElementsByTag("a");
String nextpage;
try{
nextpage = website + subpage.get(11).attr("href");
}catch(Exception e){
System.out.println("completed!");
return ;
}
loadGzrc(nextpage);
}
public static void main(String[] args) throws Exception{
GzrcService gzrc = new GzrcServiceImpl();
gzrc.loadGzrc();
}
}
<file_sep>/FigoShop/src/main/java/com/ehais/figoarticle/controller/admin/IndexAdminController.java
package com.ehais.figoarticle.controller.admin;
import java.math.BigDecimal;
import java.util.Enumeration;
import java.util.List;
import javax.script.ScriptEngine;
import javax.script.ScriptEngineManager;
import javax.script.ScriptException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.ehais.tools.EConditionObject;
import org.ehais.tools.ReturnObject;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ehais.figoarticle.controller.FigoCommonController;
import com.ehais.figoarticle.model.HaiCategory;
import com.ehais.figoarticle.model.HaiCategoryExample;
import com.ehais.figoarticle.model.HaiGoods;
import com.ehais.figoarticle.model.HaiGoodsCate;
import com.ehais.figoarticle.model.HaiWebsite;
@Controller
@RequestMapping("/shop")
public class IndexAdminController extends FigoCommonController{
@RequestMapping("/index")
public String index(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
return "/admin/main/index";
}
@RequestMapping("/cate")
public String cate(ModelMap modelMap, HttpServletRequest request, HttpServletResponse response){
List<HaiWebsite> websiteList = haiWebsiteMapper.selectByExample(null);
modelMap.addAttribute("websiteList", websiteList);
return "/admin/cate";
}
@ResponseBody
@RequestMapping("/get_cate_calculate")
public String get_cate_calculate(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "catId", required = true) Integer catId
){
ReturnObject<HaiCategory> rm = new ReturnObject<HaiCategory>();
try{
HaiCategory category = haiCategoryMapper.selectByPrimaryKey(catId);
rm.setCode(1);
rm.setModel(category);
return this.writeJson(rm);
}catch(Exception e){
e.printStackTrace();
}
return "{code:0,msg:'faild'}";
}
@ResponseBody
@RequestMapping("/save_cate_calculate")
public String save_cate_calculate(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@RequestParam(value = "catId", required = true) Integer catId,
@RequestParam(value = "calculate", required = true) String calculate
){
ReturnObject<HaiCategory> rm = new ReturnObject<HaiCategory>();
rm.setCode(0);
if(calculate.equals("")){rm.setMsg("公式不能为空");return this.writeJson(rm);}
try{
HaiCategory category = haiCategoryMapper.selectByPrimaryKey(catId);
category.setCalculate(calculate);
haiCategoryMapper.updateByPrimaryKey(category);
rm.setCode(1);
rm.setMsg("更改成功");
return this.writeJson(rm);
}catch(Exception e){
e.printStackTrace();
}
return "{code:0,msg:'faild'}";
}
@ResponseBody
@RequestMapping("/cateJson")
public String cateJson(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response,
@ModelAttribute EConditionObject eConditionObject,
@RequestParam(value = "website_id", required = false) Integer website_id,
@RequestParam(value = "emptycal", required = false) Integer emptycal,
@RequestParam(value = "cat_name", required = false) String cat_name){
Enumeration<?> temp = request.getParameterNames();
if (null != temp) {
while (temp.hasMoreElements()) {
String en = (String) temp.nextElement();
String value = request.getParameter(en);
//在报文上送时,如果字段的值为空,则不上送<下面的处理为在获取所有参数数据时,判断若值为空,则删除这个字段>
System.out.println("ParameterNames数据的键=="+en+" 值==="+value);
}
}
HaiCategoryExample example = new HaiCategoryExample();
HaiCategoryExample.Criteria c = example.createCriteria();
//c.andParentIdEqualTo(0);
if(eConditionObject.getPage() != null && eConditionObject.getRows() != null){
example.setLimitStart( eConditionObject.getStart() );
example.setLimitEnd(eConditionObject.getRows());
}
if(emptycal != null && emptycal == 1){
c.andCalculateIsNull();
}
if(cat_name != null && !cat_name.equals("")){
c.andCatNameLike("%"+cat_name+"%");
}
if(website_id != null && !website_id.equals("")){
c.andWebsiteIdEqualTo(website_id);
}
List<HaiCategory> list = haiCategoryMapper.selectByExample(example);
Long total = haiCategoryMapper.countByExample(example);
ReturnObject<HaiCategory> rm = new ReturnObject<>();
rm.setRows(list);
rm.setTotal(total);
return this.writeJson(rm);
}
@ResponseBody
@RequestMapping("/update_calculate_price")
public String update_calculate_price(ModelMap modelMap,
HttpServletRequest request,
HttpServletResponse response
){
ReturnObject<HaiCategory> rm = new ReturnObject<HaiCategory>();
rm.setCode(1);
List<HaiGoodsCate> list = haiGoodsMapper.listGoodsCate();
for (HaiGoodsCate haiGoodsCate : list) {
String calculate = haiGoodsCate.getCalculate();
calculate = calculate.replaceAll("原价", haiGoodsCate.getShopPrice().toString());
Integer balancePrice = this.calculatePrice(calculate);
haiGoodsMapper.updateBalancePrice(haiGoodsCate.getGoodsId(), balancePrice);
}
rm.setCode(1);
rm.setMsg("更新成功");
rm.setTotal(list.size());
return this.writeJson(rm);
}
public Integer calculatePrice(String calculate ) {
ScriptEngineManager manager = new ScriptEngineManager();
ScriptEngine engine = manager.getEngineByName("js");
Double result = 0d ;
try {
result = (Double)engine.eval(calculate);
} catch (ScriptException e) {
e.printStackTrace();
}
return result.intValue();
// System.out.println("结果类型:" + result.getClass().getName() + ",计算结果:" + result);
}
}
<file_sep>/pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.ehais</groupId>
<artifactId>ehproject</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>pom</packaging>
<name>ehproject</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven_compiler_ver>3.7.0</maven_compiler_ver>
<maven_resources_ver>2.7</maven_resources_ver>
<spring.version>4.3.7.RELEASE</spring.version><!-- 4.0.5.RELEASE -->
<jdk_ver>1.8</jdk_ver>
<maven_ver>3.2</maven_ver>
<junit_ver>4.11</junit_ver>
<struts2_ver>2.3.24</struts2_ver>
<logback_ver>1.0.13</logback_ver>
<servlet_api_ver>3.1.0</servlet_api_ver>
<commons_lang3_ver>3.1</commons_lang3_ver>
<springframework_ver>4.3.7.RELEASE</springframework_ver>
<slf4j_ver>1.7.5</slf4j_ver>
<jackson_ver>1.9.12</jackson_ver>
<hibernate.version>4.3.10.Final</hibernate.version>
<c3p0_ver>0.9.1.2</c3p0_ver>
<jackson.version>2.7.3</jackson.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aop</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aspects</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-beans</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context-support</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-expression</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jms</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-orm</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-oxm</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>${spring.version}</version>
<!--
<type>jar</type>
<scope>compile</scope>
-->
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<!-- -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.version}</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context-support</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-tx</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>${junit_ver}</version>
</dependency>
<!-- jackson -->
<dependency>
<groupId>org.codehaus.jackson</groupId>
<artifactId>jackson-mapper-asl</artifactId>
<version>${jackson_ver}</version>
</dependency>
<dependency>
<groupId>org.codehaus.jackson</groupId>
<artifactId>jackson-core-asl</artifactId>
<version>${jackson_ver}</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>3.0-alpha-1</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>3.13</version>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.1.3</version>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-core</artifactId>
<version>1.1.3</version>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-access</artifactId>
<version>1.1.3</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.5</version>
</dependency>
<dependency>
<groupId>opensymphony</groupId>
<artifactId>oscache</artifactId>
<version>2.4.1</version>
</dependency>
<dependency>
<groupId>com.thoughtworks.xstream</groupId>
<artifactId>xstream</artifactId>
<version>1.4.8</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.2.3</version>
</dependency>
<dependency>
<groupId>org.mybatis.generator</groupId>
<artifactId>mybatis-generator-maven-plugin</artifactId>
<version>1.3.2</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>1.2.1</version>
</dependency>
<dependency>
<groupId>net.sf.json-lib</groupId>
<artifactId>json-lib-ext-spring</artifactId>
<version>1.0.2</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-validator</artifactId>
<version>5.2.4.Final</version>
</dependency>
<dependency>
<groupId>javax.el</groupId>
<artifactId>javax.el-api</artifactId>
<version>2.2.4</version>
</dependency>
<dependency>
<groupId>org.glassfish.web</groupId>
<artifactId>javax.el</artifactId>
<version>2.2.4</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-validator-cdi</artifactId>
<version>5.2.4.Final</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-collections4</artifactId>
<version>4.1</version>
</dependency>
<dependency>
<groupId>net.sf.oval</groupId>
<artifactId>oval</artifactId>
<version>1.85</version>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.6.2</version>
</dependency>
<dependency>
<groupId>net.sourceforge.htmlunit</groupId>
<artifactId>htmlunit</artifactId>
<version>2.23</version>
</dependency>
<!--dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.1</version>
</dependency-->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.2</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpcore</artifactId>
<version>4.4.5</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpmime</artifactId>
<version>4.5.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/net.sourceforge.htmlunit/htmlunit-core-js -->
<dependency>
<groupId>net.sourceforge.htmlunit</groupId>
<artifactId>htmlunit-core-js</artifactId>
<version>2.23</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.21</version>
</dependency>
<!-- -->
<dependency>
<groupId>org.jsoup</groupId>
<artifactId>jsoup</artifactId>
<version>1.8.3</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>3.10-FINAL</version>
</dependency>
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3</version>
</dependency>
<!-- https://mvnrepository.com/artifact/javax.mail/mail -->
<dependency>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
<version>1.4</version>
</dependency>
</dependencies>
<modules>
<!--
<module>eh-weixin</module>
<module>school-weixin</module>
<module>eHR-Lucene</module>
<module>reptileV1</module>
-->
<module>eh-common</module>
<module>FigoShop</module>
</modules>
<profiles>
<profile>
<id>dev</id>
<properties>
<system.config>/META-INF/config/application-dev.properties</system.config>
</properties>
</profile>
<profile>
<id>deploy</id>
<properties>
<system.config>/META-INF/config/application-deploy.properties</system.config>
</properties>
</profile>
</profiles>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.12.4</version>
<configuration>
<skipTests>true</skipTests>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
</plugin>
</plugins>
</build>
<repositories>
<repository>
<id>jboss</id>
<url>http://repository.jboss.org/nexus/content/groups/public-jboss/</url>
<snapshots>
<enabled>true</enabled>
</snapshots>
</repository>
</repositories>
<pluginRepositories>
<pluginRepository>
<id>jboss</id>
<url>http://repository.jboss.org/nexus/content/groups/public-jboss/</url>
</pluginRepository>
</pluginRepositories>
</project><!-- <NAME> --><file_sep>/school-weixin/src/main/java/com/ehais/schoolweixin/model/wxRepair.java
package com.ehais.schoolweixin.model;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
public class wxRepair {
//手动添加日期字符串字段
private String dateStr;
public String getDateStr(){return dateStr;}
public void setDateStr(Date date){
DateFormat df=new SimpleDateFormat("yyyy-MM-dd");
dateStr=df.format(date);
}
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_repair.id
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
private Integer id;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_repair.userAddress
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
private String userAddress;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_repair.userContact
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
private String userContact;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_repair.submitDate
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
private Date submitDate;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_repair.Detail
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
private String detail;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_repair.UserWxid
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
private String userWxid;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_repair.repairClass
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
private String repairClass;
/**
* This field was generated by MyBatis Generator.
* This field corresponds to the database column wx_repair.ImageName
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
private String imageName;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_repair.id
*
* @return the value of wx_repair.id
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public Integer getId() {
return id;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_repair.id
*
* @param id the value for wx_repair.id
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public void setId(Integer id) {
this.id = id;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_repair.userAddress
*
* @return the value of wx_repair.userAddress
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public String getUserAddress() {
return userAddress;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_repair.userAddress
*
* @param userAddress the value for wx_repair.userAddress
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public void setUserAddress(String userAddress) {
this.userAddress = userAddress;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_repair.userContact
*
* @return the value of wx_repair.userContact
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public String getUserContact() {
return userContact;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_repair.userContact
*
* @param userContact the value for wx_repair.userContact
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public void setUserContact(String userContact) {
this.userContact = userContact;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_repair.submitDate
*
* @return the value of wx_repair.submitDate
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public Date getSubmitDate() {
return submitDate;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_repair.submitDate
*
* @param submitDate the value for wx_repair.submitDate
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public void setSubmitDate(Date submitDate) {
this.submitDate = submitDate;
setDateStr(submitDate);
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_repair.Detail
*
* @return the value of wx_repair.Detail
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public String getDetail() {
return detail;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_repair.Detail
*
* @param detail the value for wx_repair.Detail
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public void setDetail(String detail) {
this.detail = detail;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_repair.UserWxid
*
* @return the value of wx_repair.UserWxid
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public String getUserWxid() {
return userWxid;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_repair.UserWxid
*
* @param userWxid the value for wx_repair.UserWxid
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public void setUserWxid(String userWxid) {
this.userWxid = userWxid;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_repair.repairClass
*
* @return the value of wx_repair.repairClass
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public String getRepairClass() {
return repairClass;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_repair.repairClass
*
* @param repairClass the value for wx_repair.repairClass
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public void setRepairClass(String repairClass) {
this.repairClass = repairClass;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column wx_repair.ImageName
*
* @return the value of wx_repair.ImageName
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public String getImageName() {
return imageName;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column wx_repair.ImageName
*
* @param imageName the value for wx_repair.ImageName
*
* @mbggenerated Wed Feb 01 15:17:25 XJT 2017
*/
public void setImageName(String imageName) {
this.imageName = imageName;
}
}<file_sep>/src/main/resources/config/application-deploy.properties
jndiName=java:jboss/datasources/ehproject
| de677b48bd58aa90aef4a03a1f14b6e1a912588e | [
"SQL",
"JavaScript",
"Maven POM",
"INI",
"Java",
"Python"
] | 101 | Java | eh-project/eh-project | c96e01f1c100a0c16eba319032684c909792f656 | 7cdaa8424b8e3b6db02a4c0253c967638c997adb |
refs/heads/master | <repo_name>Grafeeva/nsudotnet<file_sep>/DatabaseApp/DatabaseApp.Data/PhilharmonicContext.cs
using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
using DatabaseApp.Data.EF;
using DatabaseApp.Data.Entities;
namespace DatabaseApp.Data {
public partial class PhilharmonicContext : DbContext {
public PhilharmonicContext ()
: base() {
//Database.SetInitializer<PhilharmonicContext>(new PhilharmonicDataBaseInitializer());
}
public virtual DbSet<Event> Event { get; set; }
public virtual DbSet<EventMember> EventMember { get; set; }
public virtual DbSet<EventType> EventType { get; set; }
public virtual DbSet<Genre> Genre { get; set; }
public virtual DbSet<Man> Man { get; set; }
public virtual DbSet<Entities.Philharmonic> Philharmonic { get; set; }
public virtual DbSet<PhilharmonicFeature> PhilharmonicFeature { get; set; }
public virtual DbSet<PhilharmonicFeatureValue> PhilharmonicFeatureValue { get; set; }
public virtual DbSet<PhilharmonicType> PhilharmonicType { get; set; }
public virtual DbSet<PhilharmonicTypeFeature> PhilharmonicTypeFeature { get; set; }
protected override void OnModelCreating ( DbModelBuilder modelBuilder ) {
modelBuilder.Entity<Event>().Property(e => e.Id);
modelBuilder.Entity<Event>().Property(e => e.IdEventType);
modelBuilder.Entity<Event>().Property(e => e.IdPhilharmonic);
modelBuilder.Entity<Event>().Property(e => e.IdImpressario);
modelBuilder.Entity<Event>().Property(e => e.IdGenre);
modelBuilder.Entity<Event>()
.HasMany(e => e.EventMembers)
.WithRequired(e => e.Event)
.HasForeignKey(e => e.IdEvent)
.WillCascadeOnDelete(true);
modelBuilder.Entity<EventMember>().Property(e => e.Id);
modelBuilder.Entity<EventMember>().Property(e => e.IdEvent);
modelBuilder.Entity<EventMember>().Property(e => e.IdArtist);
modelBuilder.Entity<EventMember>()
.Property(e => e.Reward)
.IsUnicode(false);
modelBuilder.Entity<EventType>().Property(e => e.Id);
modelBuilder.Entity<EventType>()
.Property(e => e.EventName)
.IsUnicode(false);
modelBuilder.Entity<EventType>()
.HasMany(e => e.Events)
.WithRequired(e => e.EventType)
.HasForeignKey(e => e.IdEventType)
.WillCascadeOnDelete(true);
modelBuilder.Entity<Genre>().Property(e => e.Id);
modelBuilder.Entity<Genre>()
.Property(e => e.GenreName)
.IsUnicode(false);
modelBuilder.Entity<Genre>()
.HasMany(e => e.Events)
.WithRequired(e => e.Genre)
.HasForeignKey(e => e.IdGenre)
.WillCascadeOnDelete(true);
modelBuilder.Entity<Man>().HasKey(e => e.Id);
modelBuilder.Entity<Man>()
.Property(e => e.LastName)
.IsUnicode(false);
modelBuilder.Entity<Man>()
.Property(e => e.FirstName)
.IsUnicode(false);
modelBuilder.Entity<Man>()
.Property(e => e.MiddleName)
.IsUnicode(false);
modelBuilder.Entity<Man>()
.HasMany(e => e.Events)
.WithRequired(e => e.Man)
.HasForeignKey(e => e.IdImpressario)
.WillCascadeOnDelete(true);
modelBuilder.Entity<Man>()
.HasMany(e => e.EventMembers)
.WithRequired(e => e.Man)
.HasForeignKey(e => e.IdArtist)
.WillCascadeOnDelete(false);
modelBuilder.Entity<Philharmonic>().Property(e => e.Id);
modelBuilder.Entity<Entities.Philharmonic>()
.Property(e => e.PhilharmonicName)
.IsUnicode(false);
modelBuilder.Entity<Philharmonic>().Property(e => e.IdPhilharmonicType);
modelBuilder.Entity<Philharmonic>()
.Property(e => e.Address)
.IsUnicode(false);
modelBuilder.Entity<Philharmonic>()
.HasMany(e => e.Events)
.WithRequired(e => e.Philharmonic)
.HasForeignKey(e => e.IdPhilharmonic)
.WillCascadeOnDelete(true);
modelBuilder.Entity<Philharmonic>()
.HasMany(e => e.PhilharmonicFeatureValue)
.WithRequired(e => e.Philharmonic)
.HasForeignKey(e => e.IdPhilharmonic)
.WillCascadeOnDelete(true);
modelBuilder.Entity<PhilharmonicFeature>().Property(e => e.Id);
modelBuilder.Entity<PhilharmonicFeature>()
.Property(e => e.FeatureName)
.IsUnicode(false);
modelBuilder.Entity<PhilharmonicFeature>()
.HasMany(e => e.PhilharmonicFeatureValues)
.WithRequired(e => e.PhilharmonicFeature)
.HasForeignKey(e => e.IdPhilharmonicFeature)
.WillCascadeOnDelete(true);
modelBuilder.Entity<PhilharmonicFeature>()
.HasMany(e => e.PhilharmonicTypeFeatures)
.WithRequired(e => e.PhilharmonicFeature)
.HasForeignKey(e => e.IdPhilharmonicFeature)
.WillCascadeOnDelete(true);
modelBuilder.Entity<PhilharmonicFeatureValue>().Property(e => e.Id);
modelBuilder.Entity<PhilharmonicFeatureValue>().Property(e => e.IdPhilharmonic);
modelBuilder.Entity<PhilharmonicFeatureValue>().Property(e => e.IdPhilharmonicFeature);
modelBuilder.Entity<PhilharmonicFeatureValue>()
.Property(e => e.Value)
.IsUnicode(false);
modelBuilder.Entity<PhilharmonicType>().Property(e => e.Id);
modelBuilder.Entity<PhilharmonicType>()
.Property(e => e.TypeName)
.IsUnicode(false);
modelBuilder.Entity<PhilharmonicType>()
.HasMany(e => e.PhilharmonicTypeFeatures)
.WithRequired(e => e.PhilharmonicType)
.HasForeignKey(e => e.IdPhilharmonicType)
.WillCascadeOnDelete(true);
modelBuilder.Entity<PhilharmonicType>()
.HasMany(e => e.Philharmonics)
.WithRequired(e => e.PhilharmonicType)
.HasForeignKey(e => e.IdPhilharmonicType)
.WillCascadeOnDelete(true);
modelBuilder.Entity<PhilharmonicTypeFeature>().Property(e => e.Id);
modelBuilder.Entity<PhilharmonicTypeFeature>().Property(e => e.IdPhilharmonicType);
modelBuilder.Entity<PhilharmonicTypeFeature>().Property(e => e.IdPhilharmonicFeature);
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.Data/EF/IncludeProjectionAttribute.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DatabaseApp.Data.EF {
[AttributeUsage(AttributeTargets.Class | AttributeTargets.Property)]
public class IncludeProjectionAttribute : Attribute {
public IncludeProjectionAttribute() {
}
/// <summary>
/// Создает атрибут включения
/// </summary>
/// <param name="projectionName">Название проекции</param>
public IncludeProjectionAttribute(string projectionName) {
ProjectionName = projectionName;
}
/// <summary>
/// Название проекции
/// </summary>
public string ProjectionName { get; private set; }
}
}
<file_sep>/DatabaseApp/DatabaseApp.Logic/Interfaces/ICrudService.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DatabaseApp.Data;
namespace DatabaseApp.Logic.Interfaces {
public interface ICrudService<TEntity> where TEntity : class, IEntity {
IEnumerable<TEntity> GetAll ();
IEnumerable<TEntity> GetAllByCreteria (Func<TEntity, bool> whereFunc);
TEntity GetById ( int id );
IEnumerable<TEntity> GetAllIncludeAll();
void Create ( TEntity entity );
void Update ( TEntity entity );
void Delete ( TEntity entity );
IEnumerable<TEntity> FilterCollection(Func<TEntity, bool> whereStatement);
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/PhilharmonicFeatureViewModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
namespace DatabaseApp.UI.ViewModels {
public class PhilharmonicFeatureViewModel : PropertyChangedBase {
public PhilharmonicFeatureViewModel () {
PhilharmonicFeatureEntity = new PhilharmonicFeature();
}
public PhilharmonicFeatureViewModel(PhilharmonicFeature philharmonicFeatureEntity) {
PhilharmonicFeatureEntity = philharmonicFeatureEntity;
}
public PhilharmonicFeature PhilharmonicFeatureEntity { get; private set; }
public string FeatureName {
get { return PhilharmonicFeatureEntity.FeatureName; }
set {
if (value == PhilharmonicFeatureEntity.FeatureName) return;
PhilharmonicFeatureEntity.FeatureName = value;
NotifyOfPropertyChange(() => FeatureName);
}
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/MainViewModel.cs
using System;
using System.Data;
using System.Data.Common;
using System.Linq;
using System.Windows;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
using DatabaseApp.Logic.Interfaces;
namespace DatabaseApp.UI.ViewModels {
internal class MainViewModel : PropertyChangedBase {
private IObservableCollection<PhilharmonicViewModel> _philharmonics;
public IObservableCollection<PhilharmonicViewModel> Philharmonics {
get {
return
new BindableCollection<PhilharmonicViewModel>(
_philharmonicCrudService.GetAllIncludeAll()
.Select<Philharmonic, PhilharmonicViewModel>(c => new PhilharmonicViewModel(c)));
}
set {
if (value != _philharmonics) {
_philharmonics = value;
NotifyOfPropertyChange(() => Philharmonics);
}
}
}
public IObservableCollection<EventViewModel> Events { get; set; }
public IObservableCollection<GenreViewModel> Genres { get; set; }
public IObservableCollection<EventTypeViewModel> EventTypes { get; set; }
private int _genreIndex;
public int GenreIndex {
get { return _genreIndex; }
set {
if (value != _genreIndex) {
_genreIndex = value;
if (_genreIndex != -1) {
CurrentEventViewModel.EventEntity.Genre = ManWorkspace.Genres[_genreIndex].GenreEntity;
CurrentEventViewModel.EventEntity.IdGenre = CurrentEventViewModel.EventEntity.Genre.Id;
} else {
CurrentEventViewModel.EventEntity.Genre = null;
CurrentEventViewModel.EventEntity.IdGenre = 0;
}
NotifyOfPropertyChange(() => GenreIndex);
}
}
}
private GenreViewModel _currentGenreViewModel;
public GenreViewModel CurrentGenreViewModel {
get { return _currentGenreViewModel; }
set {
if (_currentGenreViewModel != value) {
_currentGenreViewModel = value;
NotifyOfPropertyChange(() => CurrentGenreViewModel);
}
}
}
private EventViewModel _currentEventViewModel;
public EventViewModel CurrentEventViewModel {
get { return _currentEventViewModel; }
set {
if (_currentEventViewModel != value) {
_currentEventViewModel = value;
var id = _currentEventViewModel.EventEntity.IdImpressario;
ImpressarioIndex = ManWorkspace.Mans.ToList().FindIndex(v => v.ManEntity.Id == id);
id = _currentEventViewModel.EventEntity.IdEventType;
EventTypeIndex = EventTypes.ToList().FindIndex(v => v.EventTypeEntity.Id == id);
id = _currentEventViewModel.EventEntity.IdPhilharmonic;
PhilharmonicIndex = Philharmonics.ToList().FindIndex(v => v.PhilharmonicEntity.Id == id);
id = _currentEventViewModel.EventEntity.IdGenre;
GenreIndex = Genres.ToList().FindIndex(v => v.GenreEntity.Id == id);
NotifyOfPropertyChange(() => CurrentEventViewModel);
NotifyOfPropertyChange(() => CurrentEventViewModel.DateEvent);
}
}
}
private int _impressarioIndex;
public int ImpressarioIndex {
get { return _impressarioIndex; }
set {
if (value != _impressarioIndex) {
_impressarioIndex = value;
if (_impressarioIndex != -1) {
CurrentEventViewModel.Impressario = ManWorkspace.Mans[_impressarioIndex];
CurrentEventViewModel.EventEntity.IdImpressario = CurrentEventViewModel.Impressario.ManEntity.Id;
} else {
CurrentEventViewModel.Impressario = null;
CurrentEventViewModel.EventEntity.IdImpressario = 0;
}
NotifyOfPropertyChange(() => ImpressarioIndex);
}
}
}
private int _philharmonicIndex;
public int PhilharmonicIndex {
get { return _philharmonicIndex; }
set {
if (value != _philharmonicIndex) {
_philharmonicIndex = value;
if (_philharmonicIndex != -1) {
CurrentEventViewModel.Philharmonic = Philharmonics[value];
CurrentEventViewModel.EventEntity.IdPhilharmonic = CurrentEventViewModel.Philharmonic.PhilharmonicEntity.Id;
} else {
CurrentEventViewModel.Philharmonic = null;
CurrentEventViewModel.EventEntity.IdPhilharmonic = 0;
}
NotifyOfPropertyChange(() => PhilharmonicIndex);
}
}
}
private int _eventTypeIndex;
public int EventTypeIndex {
get { return _eventTypeIndex; }
set {
if (value != _eventTypeIndex) {
_eventTypeIndex = value;
if (_eventTypeIndex != -1) {
CurrentEventViewModel.EventType = EventTypes[_eventTypeIndex];
CurrentEventViewModel.EventEntity.IdEventType = CurrentEventViewModel.EventType.EventTypeEntity.Id;
} else {
CurrentEventViewModel.EventType = null;
CurrentEventViewModel.EventEntity.IdEventType = 0;
}
NotifyOfPropertyChange(() => EventTypeIndex);
}
}
}
private readonly ICrudService<Man> _manCrudService;
private readonly ICrudService<Philharmonic> _philharmonicCrudService;
private readonly ICrudService<Event> _eventCrudService;
private readonly ICrudService<Genre> _genreCrudService;
private readonly ICrudService<PhilharmonicTypeFeature> _philharmonicTypeFeatureCrudService;
private readonly ICrudService<PhilharmonicType> _philharmonicTypeCrudService;
private readonly ICrudService<PhilharmonicFeature> _philharmonicFeatureCrudService;
private readonly ICrudService<EventType> _eventTypeCrudService;
public MainViewModel(ICrudService<Man> manCrudService, ICrudService<Philharmonic> philharmonicCrudService, ICrudService<Event> eventCrudService,
ICrudService<Genre> genreCrudService, ICrudService<PhilharmonicTypeFeature> philharmonicTypeFeatureCrudService,
ICrudService<PhilharmonicType> philharmonicTypeCrudService, ICrudService<PhilharmonicFeature> philharmonicFeatureCrudService, ICrudService<EventType> eventTypeFeatureCrudService) {
_manCrudService = manCrudService;
_philharmonicCrudService = philharmonicCrudService;
_eventCrudService = eventCrudService;
_genreCrudService = genreCrudService;
_philharmonicTypeFeatureCrudService = philharmonicTypeFeatureCrudService;
_philharmonicTypeCrudService = philharmonicTypeCrudService;
_philharmonicFeatureCrudService = philharmonicFeatureCrudService;
_eventTypeCrudService = eventTypeFeatureCrudService;
Init();
}
#region Init
private void Init() {
Philharmonics = new BindableCollection<PhilharmonicViewModel>(_philharmonicCrudService.GetAllIncludeAll().Select<Philharmonic, PhilharmonicViewModel>(c => new PhilharmonicViewModel(c)));
Genres = new BindableCollection<GenreViewModel>(_genreCrudService.GetAllIncludeAll().Select<Genre, GenreViewModel>(c => new GenreViewModel(c)));
Events = new BindableCollection<EventViewModel>(_eventCrudService.GetAllIncludeAll().Select<Event, EventViewModel>(c => new EventViewModel(c)));
EventTypes = new BindableCollection<EventTypeViewModel>(_eventTypeCrudService.GetAllIncludeAll().Select<EventType, EventTypeViewModel>(c => new EventTypeViewModel(c)));
ManWorkspace = new ManWorkspaceViewModel(_manCrudService, _eventCrudService, Genres);
FeatureWorkspace = new FeatureWorkspaceViewModel(_philharmonicTypeFeatureCrudService, _philharmonicTypeCrudService, _philharmonicFeatureCrudService);
PhilharmonicWorkspace = new PhilharmonicWorkspaceViewModel(_philharmonicCrudService, FeatureWorkspace);
CurrentEventViewModel = new EventViewModel();
}
#endregion
public void NewGenre() {
CurrentGenreViewModel = new GenreViewModel();
NotifyOfPropertyChange(() => CurrentGenreViewModel);
}
public void SaveGenre() {
if (CurrentGenreViewModel.GenreEntity.Id == 0) {
_genreCrudService.Create(CurrentGenreViewModel.GenreEntity);
Genres.Add(CurrentGenreViewModel);
} else {
_genreCrudService.Update(CurrentGenreViewModel.GenreEntity);
}
CurrentGenreViewModel = new GenreViewModel();
NotifyOfPropertyChange(() => CurrentGenreViewModel);
NotifyOfPropertyChange(() => Genres);
}
public void DeleteGenre() {
if (CurrentGenreViewModel.GenreEntity.Id != 0) {
_genreCrudService.Delete(CurrentGenreViewModel.GenreEntity);
Genres.Remove(CurrentGenreViewModel);
}
CurrentGenreViewModel = new GenreViewModel();
NotifyOfPropertyChange(() => CurrentGenreViewModel);
NotifyOfPropertyChange(() => Genres);
}
public void DeleteEvent() {
if (CurrentEventViewModel.EventEntity.Id != 0) {
_eventCrudService.Delete(CurrentEventViewModel.EventEntity);
Events.Remove(CurrentEventViewModel);
}
CurrentEventViewModel = new EventViewModel();
NotifyOfPropertyChange(() => CurrentEventViewModel);
NotifyOfPropertyChange(() => Events);
}
public void NewEvent() {
CurrentEventViewModel = new EventViewModel();
NotifyOfPropertyChange(() => CurrentEventViewModel);
}
public void SaveEvent() {
try {
if (CurrentEventViewModel.EventEntity.Id == 0) {
_eventCrudService.Create(CurrentEventViewModel.EventEntity);
Events.Add(CurrentEventViewModel);
} else {
_eventCrudService.Update(CurrentEventViewModel.EventEntity);
}
CurrentEventViewModel = new EventViewModel();
NotifyOfPropertyChange(() => CurrentEventViewModel);
NotifyOfPropertyChange(() => Events);
} catch (DataException ex) {
string message = "Error.";
Exception e = ex;
while (!(e is DbException) && e.InnerException != null) {
e = e.InnerException;
}
if (e is DbException) {
message = e.Message;
}
MessageBox.Show(message);
}
}
public ManWorkspaceViewModel ManWorkspace { get; set; }
public FeatureWorkspaceViewModel FeatureWorkspace { get; set; }
public PhilharmonicWorkspaceViewModel PhilharmonicWorkspace { get; set; }
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/FeatureWorkspaceViewModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
using DatabaseApp.Logic.Interfaces;
namespace DatabaseApp.UI.ViewModels {
class FeatureWorkspaceViewModel : PropertyChangedBase {
private PhilharmonicFeatureViewModel _currentFeatureViewModel;
private PhilharmonicTypeViewModel _currentTypeViewModel;
private PhilharmonicTypeFeatureViewModel _currentTypeFeatureViewModel;
public PhilharmonicFeatureViewModel CurrentFeatureViewModel {
get { return _currentFeatureViewModel; }
set {
if (value != _currentFeatureViewModel) {
_currentFeatureViewModel = value;
NotifyOfPropertyChange(() => CurrentFeatureViewModel);
}
}
}
public PhilharmonicTypeViewModel CurrentTypeViewModel {
get { return _currentTypeViewModel; }
set {
if (value != _currentTypeViewModel) {
_currentTypeViewModel = value;
NotifyOfPropertyChange(() => CurrentTypeViewModel);
}
}
}
public PhilharmonicTypeFeatureViewModel CurrentTypeFeatureViewModel { get; set; }
public IObservableCollection<PhilharmonicTypeFeatureViewModel> PhilharmonicTypeFeatures { get; set; }
public IObservableCollection<PhilharmonicTypeViewModel> PhilharmonicTypes { get; set; }
public IObservableCollection<PhilharmonicFeatureViewModel> PhilharmonicFeatures { get; set; }
private readonly ICrudService<PhilharmonicTypeFeature> _philharmonicTypeFeatureCrudService;
private readonly ICrudService<PhilharmonicType> _philharmonicTypeCrudService;
private readonly ICrudService<PhilharmonicFeature> _philharmonicFeatureCrudService;
public FeatureWorkspaceViewModel ( ICrudService<PhilharmonicTypeFeature> philharmonicTypeFeatureCrudService,
ICrudService<PhilharmonicType> philharmonicTypeCrudService, ICrudService<PhilharmonicFeature> philharmonicFeatureCrudService ) {
_philharmonicTypeFeatureCrudService = philharmonicTypeFeatureCrudService;
_philharmonicTypeCrudService = philharmonicTypeCrudService;
_philharmonicFeatureCrudService = philharmonicFeatureCrudService;
PhilharmonicTypeFeatures = new BindableCollection<PhilharmonicTypeFeatureViewModel>(_philharmonicTypeFeatureCrudService.GetAllIncludeAll().Select<PhilharmonicTypeFeature, PhilharmonicTypeFeatureViewModel>(c => new PhilharmonicTypeFeatureViewModel(c)));
PhilharmonicTypes = new BindableCollection<PhilharmonicTypeViewModel>(_philharmonicTypeCrudService.GetAllIncludeAll().Select<PhilharmonicType, PhilharmonicTypeViewModel>(c => new PhilharmonicTypeViewModel(c)));
PhilharmonicFeatures = new BindableCollection<PhilharmonicFeatureViewModel>(_philharmonicFeatureCrudService.GetAllIncludeAll().Select<PhilharmonicFeature, PhilharmonicFeatureViewModel>(c => new PhilharmonicFeatureViewModel(c)));
CurrentFeatureViewModel = new PhilharmonicFeatureViewModel();
CurrentTypeViewModel = new PhilharmonicTypeViewModel();
CurrentTypeFeatureViewModel = new PhilharmonicTypeFeatureViewModel();
}
public void NewPhilharmonicType () {
CurrentTypeViewModel = new PhilharmonicTypeViewModel();
NotifyOfPropertyChange(() => CurrentTypeViewModel);
}
public void SavePhilharmonicType () {
if (CurrentTypeViewModel.PhilharmonicTypeEntity.Id == 0) {
_philharmonicTypeCrudService.Create(CurrentTypeViewModel.PhilharmonicTypeEntity);
PhilharmonicTypes.Add(CurrentTypeViewModel);
} else {
_philharmonicTypeCrudService.Update(CurrentTypeViewModel.PhilharmonicTypeEntity);
}
CurrentTypeViewModel = new PhilharmonicTypeViewModel();
NotifyOfPropertyChange(() => CurrentTypeViewModel);
NotifyOfPropertyChange(() => PhilharmonicTypes);
}
public void DeletePhilharmonicType () {
if (CurrentTypeViewModel.PhilharmonicTypeEntity.Id != 0) {
_philharmonicTypeCrudService.Delete(CurrentTypeViewModel.PhilharmonicTypeEntity);
PhilharmonicTypes.Remove(CurrentTypeViewModel);
}
CurrentTypeViewModel = new PhilharmonicTypeViewModel();
NotifyOfPropertyChange(() => CurrentTypeViewModel);
NotifyOfPropertyChange(() => PhilharmonicTypes);
}
}
}
<file_sep>/README.md
# nsudotnet
NSU. C# and .NET Framework
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/ManViewModel.cs
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
namespace DatabaseApp.UI.ViewModels {
class ManViewModel : PropertyChangedBase {
private ICollection<EventMemberViewModel> _eventMembers;
public ManViewModel() {
ManEntity = new Man();
}
public ManViewModel ( Man manEntity ) {
ManEntity = manEntity;
}
public Man ManEntity { get; private set; }
public string FirstName {
get { return ManEntity.FirstName; }
set {
if (value == ManEntity.FirstName) return;
ManEntity.FirstName = value;
NotifyOfPropertyChange(() => FirstName);
}
}
public string MiddleName {
get { return ManEntity.MiddleName; }
set {
if (value == ManEntity.MiddleName) return;
ManEntity.MiddleName = value;
NotifyOfPropertyChange(() => MiddleName);
}
}
public string LastName {
get { return ManEntity.LastName; }
set {
if (value == ManEntity.LastName) return;
ManEntity.LastName = value;
NotifyOfPropertyChange(() => LastName);
}
}
public DateTime? Birthdate {
get { return ManEntity.Birthdate; }
set {
if (value == ManEntity.Birthdate) return;
ManEntity.Birthdate = value;
NotifyOfPropertyChange(() => Birthdate);
}
}
public ICollection<EventMemberViewModel> EventMembers {
get {
if (_eventMembers == null) {
_eventMembers = new ObservableCollection<EventMemberViewModel>();
foreach (var member in ManEntity.EventMembers) {
_eventMembers.Add(new EventMemberViewModel(member));
}
}
return _eventMembers;
}
set {
if (!Equals(value, _eventMembers)) {
_eventMembers = value;
NotifyOfPropertyChange(() => EventMembers);
}}
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.Logic/Impl/CrudServiceImpl.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data.Common;
using System.Data.Entity;
using System.IO;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
using DatabaseApp.Data;
using DatabaseApp.Data.EF;
using DatabaseApp.Data.Entities;
using DatabaseApp.Logic.Interfaces;
namespace DatabaseApp.Logic.Impl {
public class CrudServiceImpl<TEntity> : ICrudService<TEntity> where TEntity : class, IEntity {
private DbContext _entities;
private readonly IDbSet<TEntity> _dbSet;
public CrudServiceImpl(DbContext entities) {
_entities = entities;
//_entities.Database.Log = Console.Write;
_dbSet = _entities.Set<TEntity>();
}
public IEnumerable<TEntity> GetAll() {
return _dbSet.AsEnumerable();
}
public IEnumerable<TEntity> GetAllByCreteria(Func<TEntity, bool> whereStatement) {
return FilterCollection(whereStatement);
}
public TEntity GetById(int id) {
return _dbSet.Find(id);
}
public IEnumerable<TEntity> GetAllIncludeAll() {
return _dbSet.IncludeAll().AsEnumerable();
}
public void Create(TEntity entity) {
if (entity == null) {
throw new ArgumentNullException("entity");
}
_dbSet.Add(entity);
_entities.SaveChanges();
}
public void Update(TEntity entity) {
if (entity == null) {
throw new ArgumentNullException("entity");
}
_entities.Entry(entity).State = System.Data.Entity.EntityState.Modified;
_entities.SaveChanges();
}
public void Delete(TEntity entity) {
if (entity == null) {
throw new ArgumentNullException("entity");
}
_dbSet.Remove(entity);
_entities.SaveChanges();
}
public IEnumerable<TEntity> FilterCollection(Func<TEntity, bool> whereStatement) {
return GetAllIncludeAll().Where(whereStatement).AsEnumerable();
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/AppBootstrapper.cs
using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using Autofac;
using Caliburn.Micro.Autofac;
using DatabaseApp.Data;
using DatabaseApp.Data.EF;
using DatabaseApp.Data.Entities;
using DatabaseApp.Logic.Impl;
using DatabaseApp.Logic.Interfaces;
using DatabaseApp.UI.ViewModels;
namespace DatabaseApp.UI {
class AppBootstrapper : AutofacBootstrapper<MainViewModel> {
public AppBootstrapper ()
: base() {
Initialize();
}
protected override void ConfigureContainer ( ContainerBuilder builder ) {
base.ConfigureContainer(builder);
IncludeProjectionHelper.Init(typeof(PhilharmonicContext).Assembly);
builder.RegisterType<PhilharmonicContext>().As<DbContext>().SingleInstance();
builder.RegisterType<CrudServiceImpl<Man>>().As<ICrudService<Man>>().SingleInstance();
builder.RegisterType<CrudServiceImpl<Philharmonic>>().As<ICrudService<Philharmonic>>().SingleInstance();
builder.RegisterType<CrudServiceImpl<Event>>().As<ICrudService<Event>>().SingleInstance();
builder.RegisterType<CrudServiceImpl<Genre>>().As<ICrudService<Genre>>().SingleInstance();
builder.RegisterType<CrudServiceImpl<PhilharmonicTypeFeature>>().As<ICrudService<PhilharmonicTypeFeature>>().SingleInstance();
builder.RegisterType<CrudServiceImpl<PhilharmonicType>>().As<ICrudService<PhilharmonicType>>().SingleInstance();
builder.RegisterType<CrudServiceImpl<PhilharmonicFeature>>().As<ICrudService<PhilharmonicFeature>>().SingleInstance();
builder.RegisterType<CrudServiceImpl<EventType>>().As<ICrudService<EventType>>().SingleInstance();
}
protected override void OnStartup ( object sender, StartupEventArgs e ) {
base.OnStartup(sender, e);
DisplayRootViewFor<MainViewModel>();
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.Data/EF/PhilharmonicDatabaseInitializer.cs
using System;
using System.Collections.Generic;
using System.Data.Entity;
using DatabaseApp.Data.Entities;
namespace DatabaseApp.Data.EF {
class PhilharmonicDataBaseInitializer : DropCreateDatabaseIfModelChanges<PhilharmonicContext> {
protected override void Seed ( PhilharmonicContext context ) {
base.Seed(context);
GetManList().ForEach(c => {
context.Man.Add(c);
context.SaveChanges();
});
GetPhilharmonicTypeList().ForEach(c => {
context.PhilharmonicType.Add(c);
context.SaveChanges();
});
GetPhilharmonicList(context).ForEach(c => {
context.Philharmonic.Add(c);
context.SaveChanges();
});
GetPhilharmonicFeatureList().ForEach(c => {
context.PhilharmonicFeature.Add(c);
context.SaveChanges();
});
GetPhilharmonicTypeFeatureList(context).ForEach(c => {
context.PhilharmonicTypeFeature.Add(c);
context.SaveChanges();
});
GetPhilharmonicFeatureValueList(context).ForEach(c => {
context.PhilharmonicFeatureValue.Add(c);
context.SaveChanges();
});
GetEventTypeList().ForEach(c => {
context.EventType.Add(c);
context.SaveChanges();
});
GetGenreList().ForEach(c => {
context.Genre.Add(c);
context.SaveChanges();
});
GetEventList(context).ForEach(c => {
context.Event.Add(c);
context.SaveChanges();
});
GetEventMemberList(context).ForEach(c => {
context.EventMember.Add(c);
context.SaveChanges();
});
}
private static List<Man> GetManList () {
var mans = new List<Man> {
new Man {
FirstName = "Kate",
MiddleName = "Alekseevna",
LastName = "Grafeeva",
Birthdate = new DateTime(1993, 3, 10)
},
new Man {
FirstName = "Alina",
MiddleName = "Evgenievna",
LastName = "Muravieva",
Birthdate = new DateTime(1995, 1, 19)
},
new Man() {
FirstName = "Sean",
LastName = "Bean",
Birthdate = new DateTime(1959, 4, 17)
},
new Man() {
FirstName = "Emilia",
LastName = "Clark",
Birthdate = new DateTime(1986, 10, 23)
},
new Man() {
FirstName = "Kit",
LastName = "Harington",
Birthdate = new DateTime(1986, 12, 26)
},
new Man() {
FirstName = "Sophie",
LastName = "Turner",
Birthdate = new DateTime(1996, 2, 21)
},
new Man() {
FirstName = "Peter",
LastName = "Dinklage",
Birthdate = new DateTime(1969, 6, 11)
}
};
return mans;
}
private static List<PhilharmonicType> GetPhilharmonicTypeList () {
var philharmonicTypes = new List<PhilharmonicType> {
new PhilharmonicType {
TypeName = "Theater"
},
new PhilharmonicType {
TypeName = "Opera"
},
new PhilharmonicType {
TypeName = "Cinema"
}
};
return philharmonicTypes;
}
private static List<Philharmonic> GetPhilharmonicList ( PhilharmonicContext context ) {
var philharmonics = new List<Philharmonic> {
new Philharmonic {
PhilharmonicName = "Globus",
Address = "dasda",
PhilharmonicType = context.PhilharmonicType.Find(1)
},
new Philharmonic {
PhilharmonicName = "Red Torch",
Address = "dasda",
PhilharmonicType = context.PhilharmonicType.Find(1)
},
};
return philharmonics;
}
private static List<PhilharmonicFeature> GetPhilharmonicFeatureList () {
var features = new List<PhilharmonicFeature>() {
new PhilharmonicFeature() {
FeatureName = "Capacity"
},
new PhilharmonicFeature() {
FeatureName = "Screen size"
}
};
return features;
}
private static List<PhilharmonicTypeFeature> GetPhilharmonicTypeFeatureList ( PhilharmonicContext context ) {
var typeFeatures = new List<PhilharmonicTypeFeature>() {
new PhilharmonicTypeFeature() {
PhilharmonicType = context.PhilharmonicType.Find(1),
PhilharmonicFeature = context.PhilharmonicFeature.Find(1)
},
new PhilharmonicTypeFeature() {
PhilharmonicType = context.PhilharmonicType.Find(2),
PhilharmonicFeature = context.PhilharmonicFeature.Find(1)
},
new PhilharmonicTypeFeature() {
PhilharmonicType = context.PhilharmonicType.Find(3),
PhilharmonicFeature = context.PhilharmonicFeature.Find(1)
},
new PhilharmonicTypeFeature() {
PhilharmonicType = context.PhilharmonicType.Find(2),
PhilharmonicFeature = context.PhilharmonicFeature.Find(2)
}
};
return typeFeatures;
}
private static List<PhilharmonicFeatureValue> GetPhilharmonicFeatureValueList ( PhilharmonicContext context ) {
var featureValues = new List<PhilharmonicFeatureValue>() {
new PhilharmonicFeatureValue() {
Philharmonic = context.Philharmonic.Find(1), PhilharmonicFeature = context.PhilharmonicFeature.Find(1), Value = 300.ToString()
}
};
return featureValues;
}
private static List<EventType> GetEventTypeList () {
var eventTypes = new List<EventType>() {
new EventType() {
EventName = "Concert"
},
new EventType() {
EventName = "Competition"
},
};
return eventTypes;
}
private static List<Genre> GetGenreList () {
var genres = new List<Genre> {
new Genre(){GenreName = "Comedy"},
new Genre(){GenreName = "Tragedy"}
};
return genres;
}
private static List<Event> GetEventList ( PhilharmonicContext context ) {
var events = new List<Event> {
new Event() {
EventType = context.EventType.Find(1),
Philharmonic = context.Philharmonic.Find(1),
Man = context.Man.Find(1),
Genre = context.Genre.Find(1),
DateEvent = new DateTime(2015, 05, 14)
},
new Event() {
EventType = context.EventType.Find(1),
Philharmonic = context.Philharmonic.Find(1),
Man = context.Man.Find(2),
Genre = context.Genre.Find(2),
DateEvent = new DateTime(2015, 05, 14)
}
};
return events;
}
private static List<EventMember> GetEventMemberList ( PhilharmonicContext context ) {
var eventMembers = new List<EventMember>() {
new EventMember() {
Man = context.Man.Find(1), Event = context.Event.Find(1)
},
new EventMember() {
Man = context.Man.Find(2), Event = context.Event.Find(1)
},
new EventMember() {
Man = context.Man.Find(2), Event = context.Event.Find(2)
}
};
return eventMembers;
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/PhilharmonicTypeFeatureViewModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
namespace DatabaseApp.UI.ViewModels {
public class PhilharmonicTypeFeatureViewModel:PropertyChangedBase {
public PhilharmonicTypeFeatureViewModel () {
PhilharmonicTypeFeatureEntity = new PhilharmonicTypeFeature();
}
public PhilharmonicTypeFeatureViewModel ( PhilharmonicTypeFeature philharmonicTypeFeatureEntity ) {
PhilharmonicTypeFeatureEntity = philharmonicTypeFeatureEntity;
}
public PhilharmonicTypeFeature PhilharmonicTypeFeatureEntity { get; private set; }
public string TypeName {
get { return PhilharmonicTypeFeatureEntity.PhilharmonicType.TypeName; }
set {
if (value == PhilharmonicTypeFeatureEntity.PhilharmonicType.TypeName) return;
PhilharmonicTypeFeatureEntity.PhilharmonicType.TypeName = value;
NotifyOfPropertyChange(() => TypeName);
}
}
public string FeatureName {
get { return PhilharmonicTypeFeatureEntity.PhilharmonicFeature.FeatureName; }
set {
if(PhilharmonicTypeFeatureEntity.PhilharmonicFeature.FeatureName == null) return;
PhilharmonicTypeFeatureEntity.PhilharmonicFeature.FeatureName = value;
NotifyOfPropertyChange(() => FeatureName);
}
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/PhilharmonicViewModel.cs
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
namespace DatabaseApp.UI.ViewModels {
public class PhilharmonicViewModel : PropertyChangedBase {
public PhilharmonicViewModel () {
PhilharmonicEntity = new Philharmonic();
}
public PhilharmonicViewModel ( Philharmonic philharmonicEntity ) {
PhilharmonicEntity = philharmonicEntity;
}
public Philharmonic PhilharmonicEntity { get; private set; }
public string PhilharmonicName {
get { return PhilharmonicEntity.PhilharmonicName; }
set {
if (value == PhilharmonicEntity.PhilharmonicName) return;
PhilharmonicEntity.PhilharmonicName = value;
NotifyOfPropertyChange(() => PhilharmonicName);
}
}
public string Type {
get { return PhilharmonicEntity.PhilharmonicType.TypeName; }
set {
if (value == PhilharmonicEntity.PhilharmonicType.TypeName) return;
PhilharmonicEntity.PhilharmonicType.TypeName = value;
NotifyOfPropertyChange(() => Type);
}
}
public string Address {
get { return PhilharmonicEntity.Address; }
set {
if (value == PhilharmonicEntity.Address) return;
PhilharmonicEntity.Address = value;
NotifyOfPropertyChange(() => Address);
}
}
public ICollection<FeatureValueViewModel> FeatureValue {
get {
var featureValues = new ObservableCollection<FeatureValueViewModel>();
var col = PhilharmonicEntity.PhilharmonicFeatureValue;
foreach (var c in col) {
featureValues.Add(new FeatureValueViewModel(c));
}
return featureValues;
}
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/EventViewModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
namespace DatabaseApp.UI.ViewModels {
class EventViewModel : PropertyChangedBase {
private ManViewModel _impressario;
private PhilharmonicViewModel _philharmonic;
private GenreViewModel _genre;
private EventTypeViewModel _eventType;
public EventViewModel () {
EventEntity = new Event();
}
public EventViewModel(Event eventEntity) {
EventEntity = eventEntity;
_impressario = new ManViewModel(EventEntity.Man);
_philharmonic = new PhilharmonicViewModel(EventEntity.Philharmonic);
_genre = new GenreViewModel(EventEntity.Genre);
_eventType = new EventTypeViewModel(EventEntity.EventType);
}
public Event EventEntity { get; private set; }
public string EventName {
get { return EventEntity.EventType == null ? null : EventEntity.EventType.EventName; }
set {
/*if (value == EventEntity.EventType.EventName) return;
EventEntity.EventType.EventName = value;
NotifyOfPropertyChange(() => EventName);*/
}
}
public EventTypeViewModel EventType {
get { return _eventType; }
set {
if (value == _eventType) return;
_eventType = value;
EventEntity.EventType = _eventType.EventTypeEntity;
EventEntity.IdEventType = _eventType.EventTypeEntity.Id;
NotifyOfPropertyChange(() => EventType);
NotifyOfPropertyChange(() => EventName);
}
}
public string PhilharmonicName {
get { return EventEntity.Philharmonic == null ? null : EventEntity.Philharmonic.PhilharmonicName; }
set {
/*if (value == EventEntity.Philharmonic.PhilharmonicName) return;
EventEntity.Philharmonic.PhilharmonicName = value;
NotifyOfPropertyChange(() => PhilharmonicName);*/
}
}
public PhilharmonicViewModel Philharmonic {
get { return _philharmonic; }
set {
if (value == _philharmonic) return;
_philharmonic = value;
EventEntity.Philharmonic = _philharmonic.PhilharmonicEntity;
EventEntity.IdPhilharmonic = _philharmonic.PhilharmonicEntity.Id;
NotifyOfPropertyChange(() => Philharmonic);
NotifyOfPropertyChange(() => PhilharmonicName);
}
}
public string GenreName {
get {
return EventEntity.Genre == null ? null : EventEntity.Genre.GenreName;
}
set {
/*if (value == EventEntity.Genre.GenreName) return;
EventEntity.Genre.GenreName = value;
NotifyOfPropertyChange(() => GenreName);*/
}
}
public GenreViewModel Genre {
get { return _genre; }
set {
if (value == _genre) return;
_genre = value;
EventEntity.Genre = _genre.GenreEntity;
EventEntity.IdGenre = _genre.GenreEntity.Id;
NotifyOfPropertyChange(() => Genre);
NotifyOfPropertyChange(() => GenreName);
}
}
public ManViewModel Impressario {
get { return _impressario; }
set {
if (value != _impressario) {
_impressario = value;
EventEntity.IdImpressario = _impressario.ManEntity.Id;
EventEntity.Man = _impressario.ManEntity;
NotifyOfPropertyChange(() => Impressario);
}
}
}
public DateTime? DateEvent {
get { return EventEntity.DateEvent; }
set {
if (value == EventEntity.DateEvent) return;
EventEntity.DateEvent = value;
NotifyOfPropertyChange(() => DateEvent);
}
}
public ICollection<EventMemberViewModel> EventMembers {
get {
var membersModel = new BindableCollection<EventMemberViewModel>();
var members = EventEntity.EventMembers;
foreach (var member in members) {
membersModel.Add(new EventMemberViewModel(member));
}
return membersModel; }
set {/*
if (value == EventEntity.DateEvent) return;
EventEntity.DateEvent = value;
NotifyOfPropertyChange(() => DateEvent);*/
}
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.Data/Entities/Event.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DatabaseApp.Data.EF;
namespace DatabaseApp.Data.Entities {
[IncludeProjection]
[Table("MISHKOVA12204.Event")]
public partial class Event : Entity {
public Event () {
EventMembers = new HashSet<EventMember>();
}
public DateTime? DateEvent { get; set; }
public int IdEventType { get; set; }
public int IdPhilharmonic { get; set; }
public int IdImpressario { get; set; }
public int IdGenre { get; set; }
[IncludeProjection]
public virtual EventType EventType { get; set; }
[IncludeProjection]
public virtual Philharmonic Philharmonic { get; set; }
[IncludeProjection]
public virtual Man Man { get; set; }
[IncludeProjection]
public virtual Genre Genre { get; set; }
[IncludeProjection]
public virtual ICollection<EventMember> EventMembers { get; set; }
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/PhilharmonicWorkspaceViewModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
using DatabaseApp.Logic.Interfaces;
namespace DatabaseApp.UI.ViewModels {
class PhilharmonicWorkspaceViewModel : PropertyChangedBase {
private IObservableCollection<PhilharmonicViewModel> _philharmonics;
private int _selectedFilterPhilharmonic = 0;
public int SelectedFilterPhilharmonic {
get { return _selectedFilterPhilharmonic; }
set {
if (value != _selectedFilterPhilharmonic) {
_selectedFilterPhilharmonic = value;
NotifyOfPropertyChange(() => SelectedFilterPhilharmonic);
NotifyOfPropertyChange(() => Philharmonics);
}
}
}
private int _philharmonicTypeIndex;
public int PhilharmonicTypeIndex {
get { return _philharmonicTypeIndex; }
set {
if (value != _philharmonicTypeIndex) {
_philharmonicTypeIndex = value;
NotifyOfPropertyChange(() => Philharmonics);
}
}
}
public IObservableCollection<PhilharmonicViewModel> Philharmonics {
get {
switch (_selectedFilterPhilharmonic) {
case 0:
return
new BindableCollection<PhilharmonicViewModel>(
_philharmonicCrudService.GetAllIncludeAll()
.Select<Philharmonic, PhilharmonicViewModel>(c => new PhilharmonicViewModel(c)));
case 1:
var id = FeatureWorkspace.PhilharmonicTypes[_philharmonicTypeIndex].PhilharmonicTypeEntity.Id;
return
new BindableCollection<PhilharmonicViewModel>(
_philharmonicCrudService.GetAllByCreteria(
v => v.PhilharmonicType.Philharmonics.Count(em => em.IdPhilharmonicType == id) != 0)
.Select<Philharmonic, PhilharmonicViewModel>(c => new PhilharmonicViewModel(c)));
}
return _philharmonics;
}
set {
if (value != _philharmonics) {
_philharmonics = value;
NotifyOfPropertyChange(() => Philharmonics);
}
}
}
private PhilharmonicViewModel _currentPhilharmonicViewModel;
public PhilharmonicViewModel CurrentPhilharmonicViewModel {
get { return _currentPhilharmonicViewModel; }
set {
if (_currentPhilharmonicViewModel != value) {
_currentPhilharmonicViewModel = value;
NotifyOfPropertyChange(() => CurrentPhilharmonicViewModel);
}
}
}
public void NewPhilharmonic() {
CurrentPhilharmonicViewModel = new PhilharmonicViewModel();
NotifyOfPropertyChange(() => CurrentPhilharmonicViewModel);
}
public void SavePhilharmonic() {
if (CurrentPhilharmonicViewModel.PhilharmonicEntity.Id == 0) {
_philharmonicCrudService.Create(CurrentPhilharmonicViewModel.PhilharmonicEntity);
Philharmonics.Add(CurrentPhilharmonicViewModel);
} else {
_philharmonicCrudService.Update(CurrentPhilharmonicViewModel.PhilharmonicEntity);
}
CurrentPhilharmonicViewModel = new PhilharmonicViewModel();
NotifyOfPropertyChange(() => CurrentPhilharmonicViewModel);
NotifyOfPropertyChange(() => Philharmonics);
}
public void DeletePhilharmonic() {
if (CurrentPhilharmonicViewModel.PhilharmonicEntity.Id != 0) {
_philharmonicCrudService.Delete(CurrentPhilharmonicViewModel.PhilharmonicEntity);
Philharmonics.Remove(CurrentPhilharmonicViewModel);
}
CurrentPhilharmonicViewModel = new PhilharmonicViewModel();
NotifyOfPropertyChange(() => CurrentPhilharmonicViewModel);
NotifyOfPropertyChange(() => Philharmonics);
}
private readonly ICrudService<Philharmonic> _philharmonicCrudService;
public FeatureWorkspaceViewModel FeatureWorkspace { get; private set; }
public PhilharmonicWorkspaceViewModel(ICrudService<Philharmonic> philharmonicCrudService, FeatureWorkspaceViewModel featureWorkspace) {
_philharmonicCrudService = philharmonicCrudService;
FeatureWorkspace = featureWorkspace;
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/ManWorkspaceViewModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
using DatabaseApp.Logic.Interfaces;
namespace DatabaseApp.UI.ViewModels {
internal class ManWorkspaceViewModel : PropertyChangedBase {
public IObservableCollection<GenreViewModel> Genres { get; set; }
private int _selectedFilter = 0;
public int SelectedFilter {
get { return _selectedFilter; }
set {
if (value != _selectedFilter) {
_selectedFilter = value;
NotifyOfPropertyChange(() => SelectedFilter);
NotifyOfPropertyChange(() => Mans);
}
}
}
private int _genreIndex;
public int GenreIndex {
get { return _genreIndex; }
set {
if (value != _genreIndex) {
_genreIndex = value;
NotifyOfPropertyChange(() => Mans);
}
}
}
private int _genreIndex2;
public int GenreIndex2 {
get { return _genreIndex2; }
set {
if (value != _genreIndex2) {
_genreIndex2 = value;
NotifyOfPropertyChange(() => Mans);
}
}
}
private IObservableCollection<ManViewModel> _mans;
public IObservableCollection<ManViewModel> Mans {
get {
switch (_selectedFilter) {
case 0:
return
new BindableCollection<ManViewModel>(
_manCrudService.GetAllIncludeAll().Select<Man, ManViewModel>(c => new ManViewModel(c)));
case 1:
var id = Genres[_genreIndex].GenreEntity.Id;
return
new BindableCollection<ManViewModel>(
_manCrudService.GetAllByCreteria(
v => v.EventMembers.Count(em => em.Event.IdGenre == id) != 0)
.Select<Man, ManViewModel>(c => new ManViewModel(c)));
case 2:
id = Impressarios[_impressarioIndex].ManEntity.Id;
return
new BindableCollection<ManViewModel>(
_manCrudService.GetAllByCreteria(
v => v.EventMembers.Count(em => em.Event.IdImpressario == id) != 0)
.Select<Man, ManViewModel>(c => new ManViewModel(c)));
case 3:
id = Artists[_artistIndex].ManEntity.Id;
return
new BindableCollection<ManViewModel>(
_manCrudService.GetAllByCreteria(
v => v.Events.Count(e => e.EventMembers.Count(em => em.IdArtist == id) != 0) != 0)
.Select<Man, ManViewModel>(c => new ManViewModel(c)));
case 4:
return
new BindableCollection<ManViewModel>(
_manCrudService.GetAllByCreteria(
v => v.EventMembers.Count(em => em.IdArtist == v.Id) > 1)
.Select<Man, ManViewModel>(c => new ManViewModel(c)));
case 5:
id = Genres[_genreIndex2].GenreEntity.Id;
return
new BindableCollection<ManViewModel>(
_manCrudService.GetAllByCreteria(
v => v.Events.Count(e => e.IdGenre == id) > 0)
.Select<Man, ManViewModel>(c => new ManViewModel(c)));
}
return _mans;
}
set {
if (value != _mans) {
_mans = value;
NotifyOfPropertyChange(() => Mans);
}
}
}
public IObservableCollection<ManViewModel> Artists {
get {
return
new BindableCollection<ManViewModel>(
_manCrudService.GetAllByCreteria(
v => v.EventMembers.Count() != 0)
.Select<Man, ManViewModel>(c => new ManViewModel(c)));
}
set { }
}
private int _artistIndex;
public int ArtistIndex {
get { return _artistIndex; }
set {
if (value != _artistIndex) {
_artistIndex = value;
NotifyOfPropertyChange(() => Mans);
}
}
}
public IObservableCollection<ManViewModel> Impressarios {
get {
return
new BindableCollection<ManViewModel>(
_eventCrudService.GetAll()
.Select<Event, ManViewModel>(c => new ManViewModel(c.Man)).Distinct());
}
set {
}
}
private int _impressarioIndex;
public int ImpressarioIndex {
get { return _impressarioIndex; }
set {
if (value != _impressarioIndex) {
_impressarioIndex = value;
NotifyOfPropertyChange(() => Mans);
}
}
}
private ManViewModel _currentManViewModel;
public ManViewModel CurrentManViewModel {
get { return _currentManViewModel; }
set {
if (_currentManViewModel != value) {
_currentManViewModel = value;
NotifyOfPropertyChange(() => CurrentManViewModel);
}
}
}
private readonly ICrudService<Man> _manCrudService;
private readonly ICrudService<Event> _eventCrudService;
public ManWorkspaceViewModel ( ICrudService<Man> manCrudService, ICrudService<Event> eventCrudService, IObservableCollection<GenreViewModel> genres ) {
_manCrudService = manCrudService;
_eventCrudService = eventCrudService;
Mans =
new BindableCollection<ManViewModel>(
_manCrudService.GetAllIncludeAll().Select<Man, ManViewModel>(c => new ManViewModel(c)));
Genres = genres;
}
public void NewMan() {
CurrentManViewModel = new ManViewModel();
NotifyOfPropertyChange(() => CurrentManViewModel);
}
public void SaveMan() {
if (CurrentManViewModel.ManEntity.Id == 0) {
_manCrudService.Create(CurrentManViewModel.ManEntity);
Mans.Add(CurrentManViewModel);
}
else {
_manCrudService.Update(CurrentManViewModel.ManEntity);
}
CurrentManViewModel = new ManViewModel();
NotifyOfPropertyChange(() => CurrentManViewModel);
NotifyOfPropertyChange(() => Mans);
NotifyOfPropertyChange(() => Impressarios);
NotifyOfPropertyChange(() => Artists);
}
public void DeleteMan() {
if (CurrentManViewModel.ManEntity.Id != 0) {
_manCrudService.Delete(CurrentManViewModel.ManEntity);
Mans.Remove(CurrentManViewModel);
}
CurrentManViewModel = new ManViewModel();
NotifyOfPropertyChange(() => CurrentManViewModel);
NotifyOfPropertyChange(() => Mans);
NotifyOfPropertyChange(() => Impressarios);
NotifyOfPropertyChange(() => Artists);
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.Data/Entities/PhilharmonicFeatureValue.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DatabaseApp.Data.EF;
namespace DatabaseApp.Data.Entities {
[IncludeProjection]
[Table("MISHKOVA12204.PhilharmonicFeatureValue")]
public class PhilharmonicFeatureValue : Entity {
public int IdPhilharmonic { get; set; }
public int IdPhilharmonicFeature { get; set; }
[Required]
[StringLength(100)]
public string Value { get; set; }
[IncludeProjection]
public virtual Philharmonic Philharmonic { get; set; }
[IncludeProjection]
public virtual PhilharmonicFeature PhilharmonicFeature { get; set; }
}
}
<file_sep>/DatabaseApp/DatabaseApp.Data/Entities/Philharmonic.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DatabaseApp.Data.EF;
namespace DatabaseApp.Data.Entities {
[IncludeProjection]
[Table("MISHKOVA12204.Philharmonic")]
public partial class Philharmonic : Entity {
public Philharmonic () {
Events = new HashSet<Event>();
PhilharmonicFeatureValue = new HashSet<PhilharmonicFeatureValue>();
}
[Required]
[StringLength(30)]
public string PhilharmonicName { get; set; }
public int IdPhilharmonicType { get; set; }
[Required]
[StringLength(100)]
public string Address { get; set; }
[IncludeProjection]
public virtual PhilharmonicType PhilharmonicType { get; set; }
[IncludeProjection]
public virtual ICollection<Event> Events { get; set; }
[IncludeProjection]
public virtual ICollection<PhilharmonicFeatureValue> PhilharmonicFeatureValue { get; set; }
}
}
<file_sep>/DatabaseApp/DatabaseApp.Data/Entities/EventType.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DatabaseApp.Data.EF;
namespace DatabaseApp.Data.Entities {
[IncludeProjection]
[Table("MISHKOVA12204.EventType")]
public partial class EventType : Entity {
public EventType () {
Events = new HashSet<Event>();
}
[Required]
[StringLength(30)]
public string EventName { get; set; }
[IncludeProjection]
public virtual ICollection<Event> Events { get; set; }
}
}
<file_sep>/DatabaseApp/DatabaseApp.Data/Entities/Man.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DatabaseApp.Data.EF;
namespace DatabaseApp.Data.Entities {
[IncludeProjection]
[Table("MISHKOVA12204.Man")]
public partial class Man : Entity {
public Man () {
Events = new HashSet<Event>();
EventMembers = new HashSet<EventMember>();
}
[Required]
[StringLength(30)]
public string LastName { get; set; }
[Required]
[StringLength(30)]
public string FirstName { get; set; }
[StringLength(30)]
public string MiddleName { get; set; }
public DateTime? Birthdate { get; set; }
[IncludeProjection]
public virtual ICollection<Event> Events { get; set; }
[IncludeProjection]
public virtual ICollection<EventMember> EventMembers { get; set; }
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/RadioButtonCheckedConverter.cs
using System;
using System.Collections.Generic;
using System.Globalization;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Data;
namespace DatabaseApp.UI {
public class RadioButtonCheckedConverter : IValueConverter {
public object Convert ( object value, Type targetType, object parameter, CultureInfo culture ) {
return value.Equals(System.Convert.ToInt32(parameter));
}
public object ConvertBack ( object value, Type targetType, object parameter, CultureInfo culture ) {
return System.Convert.ToBoolean(value) ? parameter : Binding.DoNothing;
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/EventTypeViewModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
namespace DatabaseApp.UI.ViewModels {
public class EventTypeViewModel: PropertyChangedBase{
public EventTypeViewModel() {
EventTypeEntity = new EventType();
}
public EventTypeViewModel (EventType eventTypeEntity) {
EventTypeEntity = eventTypeEntity;
}
public EventType EventTypeEntity { get; private set; }
public string TypeName {
get { return EventTypeEntity.EventName; }
set {
if (value != EventTypeEntity.EventName) {
EventTypeEntity.EventName = value;
NotifyOfPropertyChange(() => TypeName);
}
}
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/PhilharmonicTypeViewModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
namespace DatabaseApp.UI.ViewModels {
public class PhilharmonicTypeViewModel : PropertyChangedBase {
public PhilharmonicTypeViewModel () {
PhilharmonicTypeEntity = new PhilharmonicType();
}
public PhilharmonicTypeViewModel ( PhilharmonicType philharmonicTypeEntity ) {
PhilharmonicTypeEntity = philharmonicTypeEntity;
}
public PhilharmonicType PhilharmonicTypeEntity { get; private set; }
public string TypeName {
get { return PhilharmonicTypeEntity.TypeName; }
set {
if (value == PhilharmonicTypeEntity.TypeName) return;
PhilharmonicTypeEntity.TypeName = value;
NotifyOfPropertyChange(() => TypeName);
}
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/EventMemberViewModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
namespace DatabaseApp.UI.ViewModels {
class EventMemberViewModel : PropertyChangedBase{
private EventViewModel _event;
public EventMemberViewModel(EventMember eventMemberEntity) {
EventMemberEntity = eventMemberEntity;
_event = new EventViewModel(EventMemberEntity.Event);
}
public EventMember EventMemberEntity { get; private set; }
public ManViewModel Man {
get { return new ManViewModel(EventMemberEntity.Man); }
set {
/*ManEntity.FirstName = value;
NotifyOfPropertyChange(() => FirstName);*/
}
}
public EventViewModel Event {
get { return _event; }
set {
if (value == _event) return;
_event = value;
NotifyOfPropertyChange(() => Event);
}
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.Data/Entities/PhilharmonicTypeFeature.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DatabaseApp.Data.EF;
namespace DatabaseApp.Data.Entities {
[IncludeProjection]
[Table("MISHKOVA12204.PhilharmonicTypeFeature")]
public partial class PhilharmonicTypeFeature : Entity {
public int IdPhilharmonicType { get; set; }
public int IdPhilharmonicFeature { get; set; }
[IncludeProjection]
public virtual PhilharmonicFeature PhilharmonicFeature { get; set; }
[IncludeProjection]
public virtual PhilharmonicType PhilharmonicType { get; set; }
}
}
<file_sep>/DatabaseApp/DatabaseApp.Data/Entities/PhilharmonicType.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DatabaseApp.Data.EF;
namespace DatabaseApp.Data.Entities {
[IncludeProjection]
[Table("MISHKOVA12204.PhilharmonicType")]
public partial class PhilharmonicType : Entity {
public PhilharmonicType () {
PhilharmonicTypeFeatures = new HashSet<PhilharmonicTypeFeature>();
Philharmonics = new HashSet<Philharmonic>();
}
[Required]
[StringLength(30)]
public string TypeName { get; set; }
[IncludeProjection]
public virtual ICollection<Philharmonic> Philharmonics { get; set; }
[IncludeProjection]
public virtual ICollection<PhilharmonicTypeFeature> PhilharmonicTypeFeatures { get; set; }
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/GenreViewModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
namespace DatabaseApp.UI.ViewModels {
public class GenreViewModel: PropertyChangedBase {
public GenreViewModel () {
GenreEntity = new Genre();
}
public GenreViewModel(Genre genreEntity) {
GenreEntity = genreEntity;
}
public Genre GenreEntity { get; set; }
public string GenreName {
get { return GenreEntity.GenreName; }
set {
if(value == GenreEntity.GenreName) return;
GenreEntity.GenreName = value;
NotifyOfPropertyChange(() => GenreName);
}
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.Data/EF/IncludeProjectionHelper.cs
using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
using System.Linq.Expressions;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
namespace DatabaseApp.Data.EF {
public static class IncludeProjectionHelper {
private const string AllProjectionsKey = "__ALL";
private static readonly MethodInfo IncludeMethodInfo =
typeof(QueryableExtensions).GetMethod("Include",new[]{typeof (IQueryable<>),typeof (string)});
private static readonly Dictionary<Type, Dictionary<string, Delegate>> _cachedProjections = new Dictionary<Type, Dictionary<string, Delegate>>();
public static void Init(Assembly a) {
IEnumerable<Type> types = a.GetTypes().Where(c => c.GetCustomAttribute<IncludeProjectionAttribute>() != null);
foreach (Type type in types) {
Type queryable = typeof(IQueryable<>).MakeGenericType(type);
PropertyInfo[] members =
type
.GetProperties()
.Where(c => c.GetCustomAttributes<IncludeProjectionAttribute>().Any())
.ToArray();
var projections = new Dictionary<string, List<PropertyInfo>>();
var allProjection = new List<PropertyInfo>();
projections[AllProjectionsKey] = allProjection;
foreach (PropertyInfo memberInfo in members) {
IEnumerable<IncludeProjectionAttribute> attrs =
memberInfo.GetCustomAttributes<IncludeProjectionAttribute>();
foreach (IncludeProjectionAttribute includeProjectionAttribute in attrs) {
string key = includeProjectionAttribute.ProjectionName;
List<PropertyInfo> mil = null;
if (!string.IsNullOrWhiteSpace(key)) {
if (projections.ContainsKey(key)) mil = projections[key];
else {
mil = new List<PropertyInfo>();
projections[key] = mil;
}
}
if (mil != null) mil.Add(memberInfo);
allProjection.Add(memberInfo);
}
}
Dictionary<string, Delegate> projectionsRepo = null;
if (_cachedProjections.ContainsKey(type)) projectionsRepo = _cachedProjections[type];
else {
projectionsRepo = new Dictionary<string, Delegate>();
_cachedProjections[type] = projectionsRepo;
}
foreach (string k in projections.Keys) {
List<PropertyInfo> projectionMembers = projections[k];
ParameterExpression pexp = Expression.Parameter(queryable);
Expression source = pexp;
foreach (PropertyInfo projectionMember in projectionMembers) {
ParameterExpression o = Expression.Parameter(type);
Expression prop = Expression.Constant(projectionMember.Name);
source = Expression.Call(null, IncludeMethodInfo, source, prop);
}
LambdaExpression l = Expression.Lambda(source, pexp);
Delegate compiled = l.Compile();
projectionsRepo.Add(k, compiled);
}
}
}
public static IQueryable<TEntity> IncludeAll<TEntity>(this IQueryable<TEntity> entity) {
return IncludeProjection(entity, AllProjectionsKey);
}
public static IQueryable<TEntity> IncludeProjection<TEntity>(this IQueryable<TEntity> entity,
string projectionName) {
if (!_cachedProjections.ContainsKey(typeof(TEntity))) {
return entity;
}
Dictionary<string, Delegate> cached = _cachedProjections[typeof(TEntity)];
Delegate del = cached[projectionName];
object q = del.DynamicInvoke(entity);
return (IQueryable<TEntity>)q;
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.Data/Entities/EventMember.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DatabaseApp.Data.EF;
namespace DatabaseApp.Data.Entities {
[IncludeProjection]
[Table("MISHKOVA12204.EventMember")]
public partial class EventMember : Entity {
public int IdEvent { get; set; }
public int IdArtist { get; set; }
[StringLength(30)]
public string Reward { get; set; }
[IncludeProjection]
public virtual Event Event { get; set; }
[IncludeProjection]
public virtual Man Man { get; set; }
}
}
<file_sep>/DatabaseApp/DatabaseApp.UI/ViewModels/FeatureValueViewModel.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Caliburn.Micro;
using DatabaseApp.Data.Entities;
namespace DatabaseApp.UI.ViewModels {
public class FeatureValueViewModel: PropertyChangedBase {
public FeatureValueViewModel(PhilharmonicFeatureValue featureValueEntity) {
PhilharmonicFeatureValueEntity = featureValueEntity;
}
public PhilharmonicFeatureValue PhilharmonicFeatureValueEntity { get; private set; }
public string Value {
get { return PhilharmonicFeatureValueEntity.Value; }
set {
if(value == PhilharmonicFeatureValueEntity.Value) return;
PhilharmonicFeatureValueEntity.Value = value;
NotifyOfPropertyChange(() => Feature);
}
}
public string Feature {
get { return PhilharmonicFeatureValueEntity.PhilharmonicFeature.FeatureName; }
set {
if(value == PhilharmonicFeatureValueEntity.PhilharmonicFeature.FeatureName) return;
PhilharmonicFeatureValueEntity.PhilharmonicFeature.FeatureName = value;
NotifyOfPropertyChange(() => Value);
}
}
}
}
<file_sep>/DatabaseApp/DatabaseApp.Data/Entities/PhilharmonicFeature.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using DatabaseApp.Data.EF;
namespace DatabaseApp.Data.Entities {
[IncludeProjection]
[Table("MISHKOVA12204.PhilharmonicFeature")]
public partial class PhilharmonicFeature : Entity {
public PhilharmonicFeature () {
PhilharmonicFeatureValues = new HashSet<PhilharmonicFeatureValue>();
PhilharmonicTypeFeatures = new HashSet<PhilharmonicTypeFeature>();
}
[Required]
[StringLength(30)]
public string FeatureName { get; set; }
[IncludeProjection]
public virtual ICollection<PhilharmonicFeatureValue> PhilharmonicFeatureValues { get; set; }
[IncludeProjection]
public virtual ICollection<PhilharmonicTypeFeature> PhilharmonicTypeFeatures { get; set; }
}
}
| b240835b423e225912978e9204a4c5cd37d060c4 | [
"Markdown",
"C#"
] | 32 | C# | Grafeeva/nsudotnet | ebc2b17effa7ebe7ac47161f9012e4d94879c442 | c5bd042670642c8084f864e3d1769717e894999a |
refs/heads/main | <file_sep># coding-practise
Me working through the porblems in Cracking the Coding Interview and such.
Athir and I doing leetcode problems
1. https://leetcode.com/problems/count-substrings-that-differ-by-one-character/
2. https://leetcode.com/problems/the-k-th-lexicographical-string-of-all-happy-strings-of-length-n/
3. https://leetcode.com/problems/check-if-a-string-can-break-another-string/
4. https://leetcode.com/problems/generate-parentheses/<file_sep>from collections import defaultdict as dd
'''
1.1 Is Unique: Implement an algorithm to determine if a string has all unique
characters. What if you cannot use additional data structures?
Hints: #44, #7 7 7, #732
'''
# TODO: potential bit array solution to come back and try to implement
# TODO: implement an inplace sorting on strings??
def is_unique(string):
# basic way
# time: O(c)
# space: O(n)
# c = the number of chars in the charachter set # e.g. ascii -> c = 256
seen = set()
for i in string: # O(c)
if i in seen: # O(1)
return False
else:
seen.add(i)
return True
def is_unique2(string):
# Time: O(n)
# Space: O(n)
noDuplicates = set(string)
return len(noDuplicates) == len(string)
def is_unique3(string):
# no extra data structures
# Time: O(nlogn)
# Space: O(1)
string = sorted(string) # O(nlogn)
for i in range(len(string) - 1): # O(c)
if string[i] == string[i+1]:
return False
return True
'''
1.2 Check Permutation: Given two strings, write a method to decide if one is a
permutation of the other.
Hints: #7, #84, #722, #737
_pg 193
'''
def is_permutation1(str1, str2):
# Time: O(nlogn)
# Space: O(n)
if len(str1) != len(str2):
return False
str1 = sorted(str1)
str2 = sorted(str2)
return str1 == str2
def is_permutation(str1, str2):
# Time: O(n)
# Space: O(n)
if len(str1) != len(str2):
return False
chars1 = dd(int)
for i in str1:
chars1[i] += 1
chars2 = dd(int)
for i in str2:
chars2[i] += 1
for char in chars1:
if chars2[char] != chars1[char]:
return False
return True
'''
1.3 URLify: Write a method to replace all spaces in a string with '%20'. You may
assume that the string has sufficient space at the end to hold the additional
characters, and that you are given the "true" length of the string. (Note: If
implementing in Java, please use a character array so that you can perform this
operation in place.)
EXAMPLE
Input: "<NAME> ", 13
Output: "Mr%20John%20Smith"
Hints: #53, # 118
90
'''
def urlify(string, length):
replacement = "%20"
replace = False
for i in length:
if replace and string[i-1] == replacement[0]:
string[i] = replacement[1]
elif replace and string[i-1] == replacement[1]:
string[i] = replacement[2]
replace = False
if string[i] == ' ':
string[i] = replacement[0]
replace = True
return ''.join(string)
'''
1.4
Palindrome Permutation: Given a string, write a function to check if it is a
permutation of a palindrome. A palindrome is a word or phrase that is the same
forwards and backwards. A permutation is a rearrangement of letters. The palindrome
does not need to be limited to just dictionary words.
EXAMPLE
Input: Tact Coa
Output: True (permutations: "taco cat", "atco eta", etc.)
Hints: #106, #121, #134, #136
'''
'''
1.5
One Away: There are three types of edits that can be performed on strings: insert
a character, remove a character, or replace a character. Given two strings, write a
function to check if they are one edit (or zero edits) away.
EXAMPLE
pale, ple -> true
pales, pale -> true
pale, bale -> true
pale, bake -> false
Hints:#23, #97, #130
'''
'''
1.6
String Compression: Implement a method to perform basic string compression using the
counts of repeated characters. For example, the string aabcccccaaa would become
a2blc5a3. If the "compressed" string would not become smaller than the original string,
your method should return the original string. You can assume the string has only
uppercase and lowercase letters (a - z).
Hints:#92, #110
'''
'''
1.7 Rotate Matrix: Given an image represented by an NxN matrix, where each pixel in the
image is 4 bytes, write a method to rotate the image by 90 degrees. Can you do this in
place?
Hints: #51, # 100
'''
'''
1.8 Zero Matrix: Write an algorithm such that if an element in an MxN matrix is 0,
its entire row and column are set to 0.
Hints:#17, #74, #702
'''
'''
1.9 String Rotation:Assumeyou have a method isSubstringwhich checks if one word is a
substring of another. Given two strings, sl and s2, write code to check if s2 is a
rotation of sl using only one call to isSubstring (e.g., "waterbottle" is a rotation
of "erbottlewat").
Hints: #34, #88, # 7 04
'''<file_sep>import unittest
from ArraysStrings import *
class TestArrayStrings(unittest.TestCase):
def test_is_unique(self):
self.assertEqual(is_unique("helolol"), False)
self.assertEqual(is_unique("helo"), True)
self.assertEqual(is_unique("eelo"), False)
self.assertEqual(is_unique("eloo"), False)
def test_is_permutation(self):
self.assertEqual(is_permutation('', ''), True)
self.assertEqual(is_permutation('abc', 'cba'), True)
self.assertEqual(is_permutation('abc', 'aba'), False)
self.assertEqual(is_permutation('abc', 'cb'), False)
def test_urlify(self):
string = list("Mr <NAME> ")
self.assertEqual(urlify(string), "Mr%20John%20Smith")
if __name__ == '__main__':
unittest.main()
| 0b8eff421e396baa409a851f53705f138cc41f90 | [
"Markdown",
"Python"
] | 3 | Markdown | mariams27/coding-practise | 3bd5784385837974c0e63a1f487ff1b9ddc5204e | 216f19295ba3776e008eba55d63fcdcba8bd2d35 |
refs/heads/master | <file_sep>import rodan
__version__ = "0.0.1"
import logging
logger = logging.getLogger('rodan')
from rodan.jobs import module_loader
module_loader('rodan.jobs.neon2-wrapper.wrapper')
<file_sep># neon2-wrapper
Rodan wrapper for [Neon2](https://github.com/DDMAL/Neon2)
# Setup
Since this is a Rodan job, the first step is to set up and install [rodan-docker](https://github.com/DDMAL/rodan-docker). After that, clone this repository into
the `jobs` folder of `rodan-docker`
```
git clone --recurse-submodules https://github.com/DDMAL/neon2-wrapper
```
Neon2 is a submodule of this and tracks the develop branch. To update this, run
```
git submodule update --remote
```
from the root of `neon2-wrapper`.
Replace all instances of `demojob` with `neon2-wrapper` in `jobs/settings.py` and `docker-compose.job-dev.yml`. For more information, refer to the setup for `rodan-docker`.
# Building Neon2
You need to use webpack to build Neon2. Ensure you have [Yarn](https://yarnpkg.com) installed first. Run
```
yarn install
yarn build
```
before trying to access Neon2 in Rodan.
<file_sep>from rodan.jobs.base import RodanTask
class Neon2(RodanTask):
name = 'Neon2'
author = '<NAME> & <NAME>'
description = 'Neon Editor Online'
settings = {}
enabled = True
category = 'Pitch Correction'
interactive = True
input_port_types = [
{
'name': 'OMR',
'minimum': 1,
'maximum': 1,
'resource_types': ['application/mei+xml']
},
{
'name': 'Background',
'minimum': 1,
'maximum': 1,
'resource_types': ['image/rgba+png']
},
]
output_port_types = [
{
'name': 'Corrected',
'minimum': 1,
'maximum': 1,
'resource_types': ['application/mei+xml']
},
]
def get_my_interface(self, inputs, settings):
t = 'editor.html'
c = {
'meifile': inputs['OMR'][0]['resource_url'],
'bgimg': inputs['Background'][0]['resource_url']
}
return (t, c)
def run_my_task(self, inputs, settings, outputs):
if '@done' not in settings:
return self.WAITING_FOR_INPUT()
outfile_path = outputs['Corrected'][0]['resource_path']
outfile = open(outfile_path, 'w')
correctedMEI = settings['@user_input']
outfile.write(correctedMEI)
outfile.write("\n<!-- Corrected with Neon -->")
outfile.close()
return True
def validate_my_user_input(self, inputs, settings, user_input):
return { '@done': True, '@user_input': user_input['user_input'] }
def my_error_information(self, exc, traceback):
pass
<file_sep>{
"name": "neon2-wrapper",
"version": "1.0.0",
"main": "editor.js",
"license": "MIT",
"author": {
"name": "DDMAL",
"url": "https://ddmal.music.mcgill.ca"
},
"contributors": [
{
"name": "<NAME>",
"url": "https://github.com/zoemcl"
},
{
"name": "<NAME>",
"url": "https://github.com/JRegimbal"
}
],
"repository": {
"type": "git",
"url": "https://github.com/DDMAL/neon2-wrapper"
},
"bugs": {
"url": "https://github.com/DDMAL/neon2-wrapper/issues"
},
"scripts": {
"build": "webpack --config webpack.config.js"
},
"devDependencies": {
"bulma": "^0.7.1",
"bulma-slider": "^2.0.0",
"css-loader": "^0.28.11",
"d3": "^5.5.0",
"file-loader": "^1.1.11",
"jquery": "^3.3.1",
"style-loader": "^0.21.0",
"verovio-dev": "file:Neon2/src/verovio-dev",
"webpack": "^4.12.0",
"webpack-cli": "^3.0.8"
}
}
<file_sep>import NeonView from './Neon2/src/NeonView.js';
var view = new NeonView({
meifile: meiFile,
bgimg: bgImg,
});
$(document).ready(function () {
$('#finish-interactive').click(function () {
$.ajax({url: '', type: 'POST', data: JSON.stringify({'user_input': view.rodanGetMei()}), contentType: 'application/json', success: function () { window.close(); }});
});
});
| 8a0399250e5ee50b68dec495a2a66965488eb631 | [
"Markdown",
"JSON",
"Python",
"JavaScript"
] | 5 | Python | DDMAL/neon2-wrapper | 58d704916aefb49ec5f6ea15f6c2a5eaa483148e | 93600e30a1c65d7f95bda9347041d095b4b2bc41 |
refs/heads/master | <repo_name>Albdog/News-App<file_sep>/News/News/Controllers/DetailViewController.swift
//
// DetailViewController.swift
// News
//
// Created by <NAME> on 8/13/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
import WebKit
class DetailViewController: UIViewController, WKNavigationDelegate {
var webView: WKWebView!
var webURL: String?
override func loadView() {
super.loadView()
webView = WKWebView()
webView.navigationDelegate = self
view = webView
}
override func viewDidLoad() {
super.viewDidLoad()
if let webURL = webURL {
let url = URL(string: webURL)
webView.load(URLRequest(url: url!))
webView.allowsBackForwardNavigationGestures = true
}
}
}
<file_sep>/News/News/Controllers/NewsTableViewController.swift
//
// NewsTableViewController.swift
// News
//
// Created by <NAME> on 8/13/19.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
import Alamofire
import SwiftyJSON
class NewsTableViewController: UITableViewController {
var newsArticles = [[String:AnyObject]]()
var activityIndicatorView: UIActivityIndicatorView!
override func loadView() {
super.loadView()
activityIndicatorView = UIActivityIndicatorView(style: .gray)
tableView.backgroundView = activityIndicatorView
}
override func viewDidLoad() {
super.viewDidLoad()
loadNewsArticles { (JSON) in }
}
override func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return newsArticles.count
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
guard let cell = tableView.dequeueReusableCell(withIdentifier: "NewsViewCell", for: indexPath) as? NewsTableViewCell else {
fatalError("The dequeued cell is not an instance of NewsViewCell.")
}
var article = newsArticles[indexPath.row]
if let title = article["title"] as? String {
cell.newsTitle.text = title
} else {
cell.newsTitle.text = "No title."
}
if let urlImage = article["urlToImage"] as? String {
let url = URL(string: urlImage)
if let data = try? Data(contentsOf: url!) {
cell.newsImage.image = UIImage(data: data)
}
} else {
}
if let description = article["description"] as? String {
cell.newsDescription.text = description
} else {
cell.newsDescription.text = "No description."
}
return cell
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
guard let detailViewController = segue.destination as? DetailViewController else {
fatalError("Unexpected destination: \(segue.destination)")
}
guard let selectedNewsCell = sender as? NewsTableViewCell else {
fatalError("Unexpected sender: \(String(describing: sender))")
}
guard let indexPath = tableView.indexPath(for: selectedNewsCell) else {
fatalError("The selected cell is not being displayed by the table")
}
var article = newsArticles[indexPath.row]
if let webURL = article["url"] as? String {
detailViewController.webURL = webURL
}
}
@IBAction func reloadPressed(_ sender: Any) {
newsArticles.removeAll()
tableView.reloadData()
loadNewsArticles { (JSON) in }
}
}
extension NewsTableViewController {
func loadNewsArticles(completion: @escaping (JSON?) -> Void) {
let url = "https://newsapi.org/v2/top-headlines?country=us&category=business&apiKey=" + "3be68be40de74b5caba36f3852414f40"
activityIndicatorView.startAnimating()
tableView.separatorStyle = .none
Alamofire.request(url).responseJSON { resposeData in
guard resposeData.result.isSuccess, let value = resposeData.result.value else {
print("Error fetching news articles")
completion(nil)
return
}
let swiftyJsonVar = JSON(value)
if let resData = swiftyJsonVar["articles"].arrayObject {
self.newsArticles = resData as! [[String:AnyObject]]
}
if self.newsArticles.count > 0 {
self.activityIndicatorView.stopAnimating()
self.tableView.separatorStyle = .singleLine
self.tableView.reloadData()
}
completion(swiftyJsonVar)
}
}
}
| 60ecd7d81f7efe6e6c3045725ac78d361664e493 | [
"Swift"
] | 2 | Swift | Albdog/News-App | 7a901d7b9799fc086078ef00a8a99da4b7325c1d | f450fec43aa7138752777bf8491b8d0d88236daa |
refs/heads/master | <repo_name>roterom/lab-thinking-in-react<file_sep>/starter-code/src/components/SearchBar.js
import React, { Component } from "react";
class SearchBar extends Component {
handleChangeSearch = (e) => this.props.onSearch(e.target.value);
handleChangeCheck = (e) => this.props.onCheck(e.target.checked)
render = () => (
<div className="searchBar m-3">
<input className="form-control" type="text" placeholder="Search" value={ this.props.keyword } onChange={ this.handleChangeSearch }/>
<div className="form-group form-check">
<input type="checkbox" className="form-check-input" onChange={ this.handleChangeCheck } id="exampleCheck1"/>
<label className="form-check-label"htmlFor="exampleCheck1">Only show products in stock</label>
</div>
</div>
)
}
export default SearchBar;
<file_sep>/starter-code/src/components/FilterableProductTable.js
import React, { Component } from "react";
import SearchBar from './SearchBar';
import ProductTable from './ProductTable';
class FilterableProductTable extends Component {
state = {
search: "",
products: this.props.products.data,
onlyStocked: false
}
onSearch = (keyword) => this.setState({search: keyword});
onCheck = (status) => this.setState({onlyStocked: status});
render = () => {
const products = this.state.products
.filter(product => (this.state.onlyStocked) ? product.stocked === true: product)
.filter(product => product.name.toLowerCase().includes(this.state.search.toLocaleLowerCase()))
return (
<div>
<h1>IronStore</h1>
<SearchBar onSearch={ this.onSearch } keyword={this.state.search} onCheck={ this.onCheck }/>
<ProductTable products={ products } />
</div>
)
}
}
export default FilterableProductTable;
<file_sep>/starter-code/src/components/ProductRow.js
import React from 'react';
const ProductRow = (props) => {
const setColor = () => (!props.stocked) ? "text-danger" : null;
return (
<tr>
<td className={setColor()}>{ props.name }</td>
<td>{ props.price }</td>
</tr>
)
}
export default ProductRow;
| 1812a28cc57ff02aa1815c3a404c661677eed28c | [
"JavaScript"
] | 3 | JavaScript | roterom/lab-thinking-in-react | 119e8831bb09f38ba007ddef35bd7d0b7c222f44 | ebc122342802609b251c5f6faacaa3ebe6eaa8c2 |
refs/heads/main | <repo_name>vaibhavlanghe123/Swift-Tutorial<file_sep>/README.md
# Swift-Tutorial
The tutorial under jio training dated 22 july 2021
| 53cd7fff0f7d2e46a11caebcfcc8ff4e73f050cc | [
"Markdown"
] | 1 | Markdown | vaibhavlanghe123/Swift-Tutorial | 6509109ec44bc7dbedb5d6a26898ce1a4c615b66 | 29f721fa763e731c1a556440ffbd9671ab30f429 |
refs/heads/master | <repo_name>desenmeng/shui<file_sep>/shui.js
var request = require('https').get;
module.exports = function(domain,callback){
if(domain._ && domain._[0]){
domain = domain._[0];
}
request('https://domai.nr/api/json/info?q='+domain, function (res) {
var body = '';
res.on('data', function(chunk) {
body += chunk;
});
res.on('end', function() {
var data = JSON.parse(body)
if(typeof callback==='function'){
callback(data);
}
else{
console.log(data);
}
});
}).on('error', function(e) {
if(typeof callback==='function'){
callback(e.message);
}
else{
console.log(e.message);
}
});
};<file_sep>/demo.js
var shui = require('./shui');
shui('baidu.com',function(result){
console.log(result);
});<file_sep>/README.md
shui
====
a whois npm module
##install
```
npm install shui -g
```
##CLI
```
shui "baidu.com"
```
### Node.js
```
var shui = require('shui');
shui('baidu.com',function(result){
console.log(result);
});
```
[](https://bitdeli.com/free "Bitdeli Badge")
| 74991a88919cd400840d13d3ec7e7ff2d9c24d37 | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | desenmeng/shui | c755412c94a6e86edac11423ba9960e44d097263 | 2603035fc4a1fdbd0d3d71d27ec894910a2fb065 |
refs/heads/master | <file_sep>This is where the report generator code is kept.
<file_sep>"""
This is just a crude hack to add to the Python path.
"""
import os
import sys
sys.path.append(os.path.abspath('../lib'))
<file_sep>This is where the web spider code is kept.
<file_sep>from url import Url
from document import Document
"""
Globals to export
"""
MISSING_PAGES = ()
ALL_PAGES = ()
"""
Throw together a toy/example database of urls.
"""
urls = [
'http://spsu.edu',
'http://cse.spsu.edu',
'http://swe.spsu.edu',
'http://library.spsu.edu',
]
missing = [
'http://adfasfasf.com',
'http://foo.com/asdfasdf/wh2hjdhdf/',
'http://google.com/notarealpage/',
'http://kahsdfhasdhfjkahsf.net',
]
urls = [Document(u) for u in urls]
missing = [Document(u) for u in missing]
allp = []
allp.extend(urls)
allp.extend(missing)
for d in urls:
d.requested = True
d.httpStatus = 200
for d in missing:
d.requested = True
d.httpStatus = 404
def make_link(a, b):
if a.isMissing():
return
a.linksOut.append(b)
b.linksIn.append(a)
make_link(urls[0], urls[1])
make_link(urls[0], urls[2])
make_link(urls[0], urls[3])
make_link(urls[1], urls[0])
make_link(urls[1], urls[2])
make_link(urls[1], urls[3])
make_link(urls[2], urls[3])
make_link(urls[0], missing[0])
make_link(urls[1], missing[0])
make_link(urls[1], missing[2])
make_link(urls[2], missing[1])
make_link(urls[2], missing[2])
make_link(urls[2], missing[3])
"""
Here are the final database objects.
"""
MISSING_PAGES = tuple(allp)
ALL_PAGES = tuple(allp)
<file_sep>Forager
=======
Forager is a webcrawler for the spsu.edu domain that finds and
generates web reports on missing media files and hyperlinks
throughout the website.
This is the readme file for the project.
We will update it with more documentation as it becomes
available.
Required Python Libraries
-------------------------
* **flask** -- excellent, lightweight web platform
* **lxml** -- provides HTML parsing
* **requests** -- performs HTTP
These can be installed on Linux or Windows with `pip`,
the Python package manager, eg:
`pip install flask`
`pip install lxml`
`pip install requests`
To install lxml on Windows, you'll need the libxml2 and libxslt.
The easiest way to fix this is to simply use the precompiled
lxml found here:
* [Precompiled extensions for Windows](http://www.lfd.uci.edu/~gohlke/pythonlibs/)
<file_sep>#!/usr/bin/env python
# Python libs
import sys
import requests
import pickle
import copy
from Queue import PriorityQueue
from datetime import datetime
# Our code
import pypath
from document import Document
from url import Url
"""
CONFIGURATION
"""
ROOT_URL = Url('http://spsu.edu')
SAVE_EVERY = 7
"""
DATA STRUCTURES
Some small data structures.
"""
class RequestQueue(PriorityQueue):
def push(self, url, priority=1000):
"""Push a url onto the queue."""
self.put_nowait((priority, url))
def pop(self):
"""Pop an item from the queue."""
item = self.get()
return item[0] if len(item) == 1 else item[1]
class Database(dict):
def addUrl(self, url):
if url not in self:
self[url] = Document(url)
"""
Misc functions
"""
def save(obj, filename):
print "Saving file: %s" % filename
pickle.dump(obj, open(filename, 'wb'))
def save_database():
global DB
fn = str(datetime.now()).replace(' ', '_')
save(DB, '../cache/db_' + fn + '.obj')
def save_queue():
global RQ
# Can't pickle queue
queue = copy.copy(RQ) # XXX: Still not working
li = []
while not queue.empty():
li.append(queue.get_nowait())
fn = str(datetime.now()).replace(' ', '_')
save(li, '../cache/queue_' + fn + '.obj')
"""
GLOBALS
Some global vars for bookkeeping.
"""
# Database (dictionary of url->document) of all pages
DB = {}
# Ongoing requests
RQ = RequestQueue()
"""
MAIN
Main code.
"""
def main(url):
global DB
global RQ
doc = Document(url)
RQ.push(doc)
#DB[url] = Document(url)
try:
count = 0
while not RQ.empty():
doc = RQ.pop()
url = doc.url
print "Url '%s' dequeued." % doc.url
# Don't fetch again if in database.
if doc.url in DB:
continue
DB[url] = doc
print "Downloading..."
doc.download()
# If we just downloaded an external domain, we
# don't continue to spider it.
if not url.isOnDomain('spsu.edu'):
continue
if doc.isMissing():
continue
urls = doc.getUrls()
print "%d urls parsed from page" % len(urls)
for u in urls:
if u not in DB:
d = Document(u)
d.linksIn.append(doc)
RQ.push(d, 1) # TODO: priority heuristic
else:
d = DB[u]
d.linksIn.append(doc)
doc.linksOut.append(d)
count += 1
if count % SAVE_EVERY == 0:
save_database()
count = 1
except KeyboardInterrupt:
sys.exit()
print "Keybord Interrupt, spider terminating."
save_queue() # XXX This should be fixed.
return
except Exception as e:
import sys, traceback
print '\n---------------------'
print "Exception occurred in mainloop"
print 'Exception: %s' % e
print '- - - - - - - - - - -'
traceback.print_tb(sys.exc_info()[2])
print "\n"
pass
main(ROOT_URL)
<file_sep>This is where the web client is held.
<file_sep>"""
This module presents URL-parsing logic.
It should be able to manipulate relative and absolute URIs,
join basenames, etc.
"""
# Python stdlib
import urlparse
class Url(str):
"""
We normally don't subclass immutable strings, but we're using string
semantics and adding new functionality.
"""
def __new__(self, url, base=None):
"""
Here we join the baseUrl to the path if it's a relative url.
New is not a CTOR! New is an instance factory that fires before
the CTOR runs.
"""
absUrl = url if not base else urlparse.urljoin(base, url)
return str.__new__(self, absUrl)
def isOnDomain(self, domain):
dom = urlparse.urlparse(self)
return dom.netloc.endswith(domain)
def short(self, length=45):
"""Short representation of url"""
sht = self[0:length]
if len(sht) < len(self):
sht += '...'
return sht
# TEST CODE
if __name__ == '__main__':
u = Url("http://google.com")
print u
u = Url('../some/directory/file.gif',
'http://spsu.edu/cse/faculty')
print u
u = Url('http://google.com/some/directory/file.gif',
'http://spsu.edu/cse/faculty')
print u
<file_sep>
# Python libs
import requests
from lxml import etree
from lxml.cssselect import CSSSelector
from lxml.etree import fromstring
from StringIO import StringIO
# Our libraries
from url import Url
class Document(object):
def __init__(self, url=None):
if type(url) is str:
url = Url(url)
elif type(url) is not Url:
raise Exception, 'Must supply Url object to Document.'
self.url = url
self.title = None # Parsed out html title
self.httpStatus = None # HTTP status code
self.mimeType = None # Document type (html, jpeg, css, etc.)
self.depth = -1 # Simple depth of the page from root doc
self.requested = False # If the page has been requested
self._cachedRequest = None # Cached requests object
self._cachedLinks = [] # Cached links extracted from HTML
self.linksIn = []
self.linksOut = []
def download(self):
if self.requested:
return
self.requested = True
def parseMime(ct):
"""
Simplify/extract mimetype.
"""
if 'text/html' in ct:
return 'text/html'
return ct
try:
r = requests.get(self.url)
self.httpStatus = r.status_code
self.mimeType = parseMime(r.headers['content-type'])
self._cachedRequest = r # Cache requests object (for now)
except KeyboardInterrupt:
raise KeyboardInterrupt
except:
self.httpStatus = 0
def isMissing(self):
"""
If the HTTP status code is 404, the page is missing.
In the future, we'll consider other error codes and timeouts.
"""
return self.httpStatus in [0, 404]
def getUrls(self):
"""
Get all of the URLs in the HTML document.
If the page didn't download, we return an empty list.
The results are cached.
WORK IN PROGRESS
"""
if self._cachedLinks:
return self._cachedLinks
if self.isMissing():
return []
# TODO: Make a set rather than list to provide uniquenes
extracted = []
try:
domRoot = etree.parse(
StringIO(self._cachedRequest.content),
etree.HTMLParser()).getroot()
selector = CSSSelector('a')
links = []
for el in selector(domRoot):
if 'href' not in el.attrib:
continue
href = el.attrib['href']
# TODO: HANDLE REL LINKS
if href[0:4].lower() != 'http':
continue
url = Url(href, self.url)
links.append(url)
extracted.extend(links)
except KeyboardInterrupt:
raise KeyboardInterrupt
except:
# FIXME: Better error handling
print "Exception in DOM parsing"
self._cachedLinks = extracted
return extracted
def __getstate__(self):
"""Necessary for pickle"""
d = self.__dict__.copy()
# Unpickleable
del d['_cachedRequest']
del d['_cachedLinks']
return d
def __setstate__(self, d):
"""Necessary for pickle"""
self.__dict__.update(d)
"""
THIS IS TEST CODE --
IT'LL ONLY RUN IF THIS FILE IS EXECUTED DIRECTLY.
"""
if __name__ == '__main__':
#try:
p = Document('http://spsu.edu')
p.download()
p.getUrls()
for url in p.getUrls():
print "url: %s" % url
#except:
# print "Exception occurred"
<file_sep>This is where we keep misc logs, etc.
<file_sep>#!/usr/bin/env python
"""
This is the flask website that powers report viewing.
"""
# Python libs
import os
import glob
import random
from flask import Flask
from flask import render_template
# Our code
import pypath
import pickle
#from database import ALL_PAGES, MISSING_PAGES
from document import Document
from url import Url
"""
THIS IS REALLY MESSY INTEGRATION!
The reports are compiled here.
Bad Brandon! Bad!
"""
CACHE_DIR = '../cache'
dirfiles = glob.glob(CACHE_DIR + '/*obj')
dirfiles.sort()
DATABASE_FILE = dirfiles[-2]
f = open(DATABASE_FILE)
db = pickle.loads(f.read())
pagelist = []
i = 0
for url, doc in db.iteritems():
doc.cacheId = i
pagelist.append(doc)
i+= 1
ALL_PAGES = tuple(pagelist)
errlist = []
for doc in ALL_PAGES:
if doc.isMissing():
errlist.append(doc)
# Generate page statistics
for doc in ALL_PAGES:
outBad = 0
for d in doc.linksOut:
if d.isMissing():
outBad += 1
doc.cacheOutBad = outBad
MISSING_PAGES = tuple(errlist)
"""
FLASK APP.
Flask rocks for fast web backend dev.
"""
app = Flask(__name__)
@app.route('/')
def page_index():
global ALL_PAGES, MISSING_PAGES
return render_template('index.html', all=ALL_PAGES, missing=MISSING_PAGES)
@app.route('/url/<urlId>')
@app.route('/url/<urlId>/')
def page_report(urlId):
global ALL_PAGES
return render_template('url.html', doc=ALL_PAGES[int(urlId)])
if __name__ == "__main__":
app.run()
| cec8c122014787dfc445e3c78949124e920ab539 | [
"Markdown",
"Python"
] | 11 | Markdown | spsu/forager | fb6ecd4199a0962782aa01a589ac3770b0cd0c63 | 5250487ec32837bd64e42b1879177eabc80b9e69 |
refs/heads/master | <repo_name>sachioross/roadmap<file_sep>/scripts/roadmap.js
var app = angular.module("XK_Roadmap", []);
var trello = {
boardsUrl: "https://trello.com/1/boards/",
listsUrl: "https://trello.com/1/lists/",
bedrockBoardId: "54401ff4d3f56f85f5daeadb",
queryParamMark: "?",
queryParamConcat: "&",
key: "key=<KEY>",
token: "token=<KEY>",
cardsAllParam: "cards=all",
listAllParam: "lists=all",
writeToken: "token=<KEY>",
requestedListId: "54a360f7f59a9f0bedce16f9",
keyValue: "<KEY>",
tokenValue: "<KEY>",
writeTokenValue: "<KEY>"
}
trello.keyAndToken = trello.key + trello.queryParamConcat + trello.token;
trello.keyAndWriteToken = trello.key + trello.queryParamConcat + trello.writeToken;
function concat() {
var concatenated = "";
for(i in arguments) {
concatenated += arguments[i];
}
return concatenated;
}
function createPromiseSuccessForIndex($scope, promise, index) {
promise.success(function(data, status){
$scope.lists[index].cards = data;
})
}
app.controller("RoadmapBoard", ['$http', '$scope', function($http, $scope) {
var trelloBoardAddress = concat(trello.boardsUrl,
trello.bedrockBoardId,
trello.queryParamMark,
trello.keyAndToken,
trello.queryParamConcat,
trello.cardsAllParam,
trello.queryParamConcat,
trello.listAllParam);
$http.get(trelloBoardAddress)
.success(function(data, status){
$scope.lists = new Array();
for(i in data.lists) {
if (data.lists[i].name !== "Requested") {
$scope.lists.push(data.lists[i]);
} else {
$scope.requested = data.lists[i];
}
}
$http.get(concat(trello.listsUrl,
$scope.requested.id,
"/cards",
trello.queryParamMark,
trello.keyAndToken))
.success(function(data, status) {
$scope.requested.cards = data;
console.log($scope.requested.cards);
})
.error(function(data, status) {
console.log("Error while fetching requested cards: " + status);
});
for (var i in $scope.lists) {
var promise = $http.get(concat(trello.listsUrl,
$scope.lists[i].id,
"/cards",
trello.queryParamMark,
trello.keyAndToken))
.error(function(data, status) {
console.log("error: " + status);
});
createPromiseSuccessForIndex($scope, promise, i);
}
})
.error(function(data, status){
console.log("error: " + status);
});
}])
.controller("RegisterController", ['$scope', '$http', function($scope, $http) {
$scope.register = function() {
var trelloWriteListAddress = concat(trello.listsUrl,
trello.requestedListId,
"/cards",
trello.queryParamMark,
trello.keyAndWriteToken,
trello.queryParamConcat,
"name=",
$scope.feature.title,
trello.queryParamConcat,
"desc=",
$scope.feature.description,
trello.queryParamConcat,
"due=null");
var trelloWriteData = {
key: trello.keyValue,
token: trello.writeTokenValue,
name: $scope.feature.title,
description: $scope.feature.description,
due: "null"
}
var req = {
method: 'POST',
url: trelloWriteListAddress,
headers: {
'Content-Type': undefined
},
data: trelloWriteData
}
$http(req)
.success(function(data, status) {
$('.standard.addcard-success').modal('show');
})
.error(function(data, status) {
$('.standard.addcard-failure').modal('show');
});
};
}]);<file_sep>/scripts/xms-incident-client.js
var incidentFormValidationRules = {
ClientName: {
identifier: "ClientName",
rules: [{
type: 'empty',
prompt: 'Choose a client'
}]
},
ClientContact: {
identifier: "ClientContact",
rules: [{
type: 'empty',
prompt: 'Please provide the name of the person from the client that we should contact'
}]
},
ClientPhone: {
identifier: "ClientPhone",
rules: [{
type: 'empty',
prompt: 'Please enter a phone number for the person mentioned above'
}]
},
IncidentDescription: {
identifier: "IncidentDescription",
rules: [{
type: 'empty',
prompt: 'Please enter a description of the incident as told by the client'
}]
}
}
$(document)
.ready(function() {
$('.standard.support.modal').modal();
$('.ui.form').form(incidentFormValidationRules, {
on: 'blur',
inline: 'true'
});
});
<file_sep>/scripts/site.js
var cardFormValidationRules = {
CardName: {
identifier: "CardName",
rules: [{
type: 'empty',
prompt: 'Please enter a concise title for the request'
}]
},
Description: {
identifier: "CardDescription",
rules: [{
type: 'empty',
prompt: 'Please provide a detailed description for the team!'
}]
}
}
$(document)
.ready(function() {
$('.addcard.modal').modal({allowMultiple: true});
$('.standard.addcard.modal').modal('attach events', '.addcard.button');
$('.ui.form').form(cardFormValidationRules, {
on: 'blur',
inline: 'true'
});
$('.requested-features.modal').modal('attach events', '.requestedfeatures.button');
});
<file_sep>/scripts/xms.js
var app = angular.module("XK_XMS", []);
var xms = {};
app.controller("IncidentForm", ['$http', '$scope', function($http, $scope) {
$scope.clients = getClientList();
$scope.reportIncident = function() {
$http.post('/submit/incident', $scope.incident)
.success(function(data, status) {
$('.standard.support.modal').modal('show');
})
.error(function(data, status) {
console.log(status + " :( " + data);
});
};
$scope.select = function(selected) {
$scope.incident = $scope.incident || {};
$scope.incident.client = selected.id;
};
}]);
app.controller("SupportNumberController", ['$http', '$scope', function($http, $scope) {
$scope.people = getSupportList();
}])
$(document).ready(function(){
$('.ui.dropdown').dropdown();
});
function getClientList() {
// TODO: This is the function to implement when we have a client-hosting system
return xms.dev.clients;
}
function getSupportList() {
// TODO: This is the function to implement when we have a client-hosting system
return xms.dev.supportList;
}
/* FOR USE DURING DEVELOPMENT ONLY */
xms.dev = {};
xms.dev.clients = [
{
name: "LATAM",
id: "latam"
},
{
name: "Citrix",
id: "citrix"
},
{
name: "ClearPath",
id: "clearpath"
},
{
name: "Digicel",
id: "digicel"
}
];
xms.dev.supportList = [
{
name: "Chepe",
phone: "1234-5678",
skype: "chepe.xumak"
},
{
name: "Federico",
phone: "1234-5678",
skype: "federico.xumak"
},
{
name: "Dulio",
phone: "1234-5678",
skype: "dulio.xumak"
}
]
| 0f6e592acacb7afb0408670a83cd179498dab37d | [
"JavaScript"
] | 4 | JavaScript | sachioross/roadmap | 0d9ef90169ac9d5fe2900523864b540071ddbdee | c6e5e712b35cb18708bb91d112f982f1b13b686c |
refs/heads/master | <file_sep>import React, { Component } from 'react';
class NameChange extends Component {
render() {
return (
<div className="message system">
{this.props.currentuser} changed their name to {this.props.username}.
</div>
);
}
}
export default NameChange;<file_sep>import React, { Component } from 'react';
import Message from './Message.jsx';
import NameChange from './NameChange.jsx';
class List extends Component {
render() {
//console.log(`MessageList.List`);
// TODO - check for a message length - if no length return back
console.log(this.props.messages);
const messages = this.props.messages.map(message => {
if (message.type == "incomingNotification") {
return <NameChange
key={message.id}
username={message.username}
currentuser={message.currentUser}
/>
} else if (message.type == "incomingMessage") {
return < Message
key={message.id}
type={message.type}
username={message.username}
content={message.content}
color={message.color}
//messages={message}
/>
}
})
return (
<main className="messages">
{messages}
</main>
);
}
}
export default List;<file_sep># Chatty
=====================
## Description
Chatty is a single page multi user messaging app.
#Final Product



## Getting Started
1. Fork this repository
<EMAIL>:https://github.com/nvngsys/chattyapp.git
2. Clone your fork of this repository.
3. Install dependencies using the `npm install` command.
- from main project directory run 'npm install'
- cd to chatty_server and run 'npm install'
4. Start the websocket server
- CD to chatty_server in a separate terminal tab
- Start server using 'npm start'
- The web socket server will run on <http://localhost:3001/>.
5. Start the web server
- cd to main project directory.
- start web server 'npm start'
- Go to <http://localhost:3000/> in your browser.
### Dependencies
* React
* Webpack
* [babel-loader](https://github.com/babel/babel-loader)
* [webpack-dev-server](https://github.com/webpack/webpack-dev-server)
| 995c0a0505b0d751a2734cf9b55336d28428ad00 | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | nvngsys/chattyapp | 74940abea4db069e9bac88138814c962d657395b | 92805a2e598925c0b7894efb8ff055d51376312f |
refs/heads/master | <repo_name>jbv2/nf-join-beds<file_sep>/run_test.sh
nextflow join_beds.nf --input_dir ./test/data --chunks 2 -resume
<file_sep>/mkmodules/mk-tag-filename/mkfile
MKSHELL=/bin/bash
#Definir MKSHELL ahora debe ir siempre arriba con /bin/bash. "Todo lo que viene a continuación interprétalo con bash"
#Este bloque simplifica el vcf con bcftools para hacerlo más fácilmente manipulable
%.tagged.bed:Q: %.bed
echo "Adding file name as las column for $prereq"
awk '
function basename(file, a, n) {
n = split(file, a, "/")
return a[n]
}
{print $0, basename(FILENAME)}
' $prereq > $target.build \
&& mv $target.build $target
<file_sep>/README.md
# nf-join-beds
A pipeline to join and tag beds from different files.
test data is a sample from:
http://www.targetscan.org/vert_72/vert_72_data_download/All_Target_Locations.hg19.bed.zip
<file_sep>/mkmodules/mk-concatenate-beds/mkfile
MKSHELL=/bin/bash
#Definir MKSHELL ahora debe ir siempre arriba con /bin/bash. "Todo lo que viene a continuación interprétalo con bash"
#Este bloque simplifica el vcf con bcftools para hacerlo más fácilmente manipulable
%/tagged.sorted.concatenated.bed:Q: %/
echo "[DEBUG] Concatenando los archivos BEDs que radican en $prereq ; y que son $(ls $prereq)"
bedops -u $prereq*.bed > $target
<file_sep>/mkmodules/mk-concatenate-beds/runmk.sh
#!/bin/bash
find -L . \
-type f \
-name "*.sorted.bed" \
! -name "tagged.sorted.concatenated.bed" \
-exec dirname {} \; \
| sort -u \
| sed "s#\$#/tagged.sorted.concatenated.bed#" \
| xargs mk $@
<file_sep>/mkmodules/mk-sort-bed/mkfile
MKSHELL=/bin/bash
#Definir MKSHELL ahora debe ir siempre arriba con /bin/bash. "Todo lo que viene a continuación interprétalo con bash"
#Este bloque simplifica el vcf con bcftools para hacerlo más fácilmente manipulable
%.sorted.bed:Q: %.bed
echo "Sorting BED files $prereq"
cat $prereq \
| sort -k1,1 -k2,2n $prereq > $target
| 7499b364564b02408664182c33a3cba48297ad33 | [
"Markdown",
"Makefile",
"Shell"
] | 6 | Shell | jbv2/nf-join-beds | 2335ee5398e2a469752741a28022720563222958 | 18d889f484c235942df3cbc5518a8122d907e783 |
refs/heads/master | <file_sep>$(document).ready(function(){
$("#d").click(function(){
$("#d").toggleClass("red");
});
$("#di").click(function(){
$("#di").toggleClass("blue");
});
$("#dii").click(function(){
$("#dii").toggleClass("green");
});
});
<file_sep>$(document).ready(function(){
$("h1").click(function(){
$("#myDIV").toggle();
});
}); | dfd0d53f398496e2ded8f3fc5de04458bb119264 | [
"JavaScript"
] | 2 | JavaScript | nikitha11/jquery | 2a59e189da9565c712f1464c37dc7b42c04317d4 | 53b55bd64ccdb5eb016bfd152369e96b40761695 |
refs/heads/master | <repo_name>HusnaJaved241/djangorestapi<file_sep>/students/viewsets.py
from rest_framework import viewsets
from . import models
from . import serializers
class StudentsViewset(viewsets.ModelViewSet):
queryset = models.Students.objects.all()
serializer_class = serializers.StudentsSerializer<file_sep>/restapi/router.py
from students.viewsets import StudentsViewset
from rest_framework import routers
router = routers.DefaultRouter()
router.register('students',StudentsViewset)
# localhost:p/api/employee/5
# GET, POST, PUT, DELETE
# list , retrieve | a7571352d10ce93307471679d2f14510a6477b55 | [
"Python"
] | 2 | Python | HusnaJaved241/djangorestapi | 564d2814bc855a78327f8cb4e191a39b08df6184 | bdc96b2f98bb4d306ffe1fdb6ac2fc6a13c250bb |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.InteropServices;
using System.Text;
using System.Threading.Tasks;
namespace Clifton.Utils
{
public static class ConsoleWindowPosition
{
const int SWP_NOSIZE = 0x0001;
[System.Runtime.InteropServices.DllImport("kernel32.dll")]
private static extern bool AllocConsole();
[DllImport("user32.dll", EntryPoint = "SetWindowPos")]
public static extern IntPtr SetWindowPos(IntPtr hWnd, int hWndInsertAfter, int x, int Y, int cx, int cy, int wFlags);
[DllImport("kernel32.dll", SetLastError = true)]
public static extern IntPtr GetConsoleWindow();
// http://stackoverflow.com/questions/1548838/setting-position-of-a-console-window-opened-in-a-winforms-app/1548881#1548881
public static void SetConsoleWindowPosition(int x, int y)
{
AllocConsole();
IntPtr MyConsole = GetConsoleWindow();
SetWindowPos(MyConsole, 0, x, y, 0, 0, SWP_NOSIZE);
}
}
}
<file_sep>/*
Copyright (c) 2015, <NAME>
All rights reserved.
Article: http://www.codeproject.com/Articles/859108/Writing-a-Web-Server-from-Scratch
Git: https://github.com/cliftonm/BasicWebServer.git
Redistribution and use in source and binary forms, with or without modification,
are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice, this list
of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice, this
list of conditions and the following disclaimer in the documentation and/or other
materials provided with the distribution.
* Neither the name of MyXaml nor the names of its contributors may be
used to endorse or promote products derived from this software without specific
prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR
ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON
ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Text;
using System.Threading.Tasks;
using Clifton.Extensions;
using Clifton.Utils;
namespace Clifton.WebServer
{
/// <summary>
/// Sessions are associated with the client IP.
/// </summary>
public class Session
{
public DateTime LastConnection { get; set; }
/// <summary>
/// Is the user authenticated?
/// </summary>
public bool Authenticated { get; set; }
/// <summary>
/// Can be used by controllers to add additional information that needs to persist in the session.
/// </summary>
private Dictionary<string, object> Objects { get; set; }
// Indexer for accessing session objects. If an object isn't found, null is returned.
public object this[string objectKey]
{
get
{
object val=null;
Objects.TryGetValue(objectKey, out val);
return val;
}
set { Objects[objectKey] = value; }
}
/// <summary>
/// Object collection getter with type conversion.
/// Note that if the object does not exist in the session, the default value is returned.
/// Therefore, session objects like "isAdmin" or "isAuthenticated" should always be true for their "yes" state.
/// </summary>
public T GetObject<T>(string objectKey)
{
object val = null;
T ret = default(T);
if (Objects.TryGetValue(objectKey, out val))
{
ret = (T)Converter.Convert(val, typeof(T));
}
return ret;
}
public Session()
{
Objects = new Dictionary<string, object>();
UpdateLastConnectionTime();
}
public void UpdateLastConnectionTime()
{
LastConnection = DateTime.Now;
}
/// <summary>
/// Returns true if the last request exceeds the specified expiration time in seconds.
/// </summary>
public bool IsExpired(int expirationInSeconds)
{
return (DateTime.Now - LastConnection).TotalSeconds > expirationInSeconds;
}
/// <summary>
/// De-authorize the session.
/// </summary>
public void Expire()
{
Authenticated = false;
// Don't remove the validation token, as we still essentially have a session, we just want the user to log in again.
}
}
public class SessionManager
{
protected Server server;
/// <summary>
/// Track all sessions.
/// </summary>
protected Dictionary<IPAddress, Session> sessionMap = new Dictionary<IPAddress, Session>();
// TODO: We need a way to remove very old sessions so that the server doesn't accumulate thousands of stale endpoints.
public SessionManager(Server server)
{
this.server = server;
sessionMap = new Dictionary<IPAddress, Session>();
}
/// <summary>
/// Creates or returns the existing session for this remote endpoint.
/// </summary>
public Session GetSession(IPEndPoint remoteEndPoint)
{
Session session;
if (!sessionMap.TryGetValue(remoteEndPoint.Address, out session))
{
session=new Session();
session[server.ValidationTokenName] = Guid.NewGuid().ToString();
sessionMap[remoteEndPoint.Address] = session;
}
return session;
}
}
}
<file_sep>BasicWebServer
==============
A lean and mean web server implemented in C#
<file_sep>/*
Copyright (c) 2015, <NAME>
All rights reserved.
Article: http://www.codeproject.com/Articles/859108/Writing-a-Web-Server-from-Scratch
Git: https://github.com/cliftonm/BasicWebServer.git
Redistribution and use in source and binary forms, with or without modification,
are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice, this list
of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice, this
list of conditions and the following disclaimer in the documentation and/or other
materials provided with the distribution.
* Neither the name of MyXaml nor the names of its contributors may be
used to endorse or promote products derived from this software without specific
prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR
ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON
ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Clifton.Extensions;
namespace Clifton.WebServer
{
/// <summary>
/// The base class for route handlers. If not for being abstract, this would be the equivalent of an anonymous handler,
/// but we want to enforce an explicit declaration of that so the developer doesn't accidentally use RouteHandler without
/// realizing that it's an anonymous, unauthenticated, no session timeout check, handler. Defensive Programming!
/// </summary>
public abstract class RouteHandler
{
protected Server server;
protected Func<Session, Dictionary<string, object>, ResponsePacket> handler;
public RouteHandler(Server server, Func<Session, Dictionary<string, object>, ResponsePacket> handler)
{
this.server = server;
this.handler = handler;
}
public virtual ResponsePacket Handle(Session session, Dictionary<string, object> parms)
{
return InvokeHandler(session, parms);
}
/// <summary>
/// CanHandle is used only for determining which handler, in a multiple handler for a single route, can actually handle to session and params for that route.
/// </summary>
public virtual bool CanHandle(Session session, Dictionary<string, object> parms) { return true; }
protected ResponsePacket InvokeHandler(Session session, Dictionary<string, object> parms)
{
ResponsePacket ret = null;
handler.IfNotNull((h) => ret = h(session, parms));
return ret;
}
}
/// <summary>
/// Page is always visible.
/// </summary>
public class AnonymousRouteHandler : RouteHandler
{
public AnonymousRouteHandler(Server server, Func<Session, Dictionary<string, object>, ResponsePacket> handler = null)
: base(server, handler)
{
}
}
/// <summary>
/// Page is visible only to authorized users.
/// </summary>
public class AuthenticatedRouteHandler : RouteHandler
{
public AuthenticatedRouteHandler(Server server, Func<Session, Dictionary<string, object>, ResponsePacket> handler = null)
: base(server, handler)
{
}
public override ResponsePacket Handle(Session session, Dictionary<string, object> parms)
{
ResponsePacket ret;
if (session.Authenticated)
{
ret = InvokeHandler(session, parms);
}
else
{
ret = server.Redirect(server.OnError(Server.ServerError.NotAuthorized));
}
return ret;
}
}
/// <summary>
/// Page is visible only to authorized users whose session has not expired.
/// </summary>
public class AuthenticatedExpirableRouteHandler : AuthenticatedRouteHandler
{
public AuthenticatedExpirableRouteHandler(Server server, Func<Session, Dictionary<string, object>, ResponsePacket> handler = null)
: base(server, handler)
{
}
public override ResponsePacket Handle(Session session, Dictionary<string, object> parms)
{
ResponsePacket ret;
if (session.IsExpired(server.ExpirationTimeSeconds))
{
session.Expire();
ret = server.Redirect(server.OnError(Server.ServerError.ExpiredSession));
}
else
{
ret = base.Handle(session, parms);
}
return ret;
}
}
}
| 4abdba0130979c16610367aaface2cc657cce37b | [
"Markdown",
"C#"
] | 4 | C# | 3wayHimself/BasicWebServer | 0768f6c077c27bd3761acf295476df1acc48caf5 | 5c8f3e130910d13d25d7a9c851ea777748b60a9b |
refs/heads/master | <file_sep># Image Compression and Decompression System
Model is trained on https://www.kaggle.com/nikhilpandey360/chest-xray-masks-and-labels dataset.
We have preprocessed the data by running 'preprocess.py'.
It will convert all images into specific dimensions.
'train/*' means all training images are placed in the 'train' folder.
For training the model,we have converted the dataset into .h5 file.
To convert into .h5 file, I have uploaded createh5.py file.
Please change the name respective of .h5 file name in config.py
Model is trained by running the command -
python3 train.py -opt momentum --name my_network
Pretrained Model -
https://drive.google.com/file/d/1RDoVQmxNbJESOBkmXH6T_3T811Zq0K2s/view?usp=sharing
The model is trained on 60 epochs with diagnostic-step size as 30.
For Compressing and Decompressing the image we will run the command -
python3 compress.py -rl -i input_image_path -o random_output_image_name
For deployment of the model -
After training the model download the checkpoint and save it in the directory where app.py is stored.
Run command -
python app.py
Go to browser and type http://localhost:5000/ to get the view of the system.
For uploading image you should upload the preproccesed image.
Output image will be stored in the 'output' folder.
Hope you will enjoy it!!!
# RVPS_Image_Compression_GAN
<file_sep>import glob
import pandas as pd
a=[]
for name in glob.glob('train/*'):
a.append(name[6:])
initial_data = {}
df = pd.DataFrame(initial_data, columns = [])
df["path"]=a
print(df)
import pandas as pd
hf = pd.HDFStore('mydata.h5')
hf.put('df',df)
hf.close()
ab = pd.HDFStore('mydata.h5',mode='r')
print(ab.keys())
df1 = ab.get('df')
print(df1)
<file_sep>import tensorflow.compat.v1 as tf
tf.disable_v2_behavior()
import numpy as np
import pandas as pd
import time, os, sys
import argparse
import json;
# User-defined
from network import Network
from utils import Utils
from data import Data
from model import Model
from config import config_test, directories
import os
from flask import Flask, render_template,jsonify, request,send_from_directory,flash,redirect, url_for
from werkzeug import secure_filename
from pathlib import Path
import hashlib
import sys
tf.logging.set_verbosity(tf.logging.ERROR)
UPLOAD_FOLDER = 'upload_input/'
OUTPUT_FOLDER = 'output/final.png'
ALLOWED_EXTENSIONS = {'txt', 'pdf', 'png', 'jpg', 'jpeg', 'gif'}
app = Flask(__name__)
app.config['UPLOAD_FOLDER'] = UPLOAD_FOLDER
app.config['OUTPUT_FOLDER'] = OUTPUT_FOLDER
def single_compress(config, args):
start = time.time()
ckpt = tf.train.get_checkpoint_state(directories.checkpoints)
assert (ckpt.model_checkpoint_path), 'Missing checkpoint file!'
if config.use_conditional_GAN:
print('Using conditional GAN')
paths, semantic_map_paths = np.array([args.image_path]), np.array([args.semantic_map_path])
else:
paths = np.array([args.image_path])
gan = Model(config, paths, name='single_compress', dataset=args.dataset, evaluate=True)
saver = tf.train.Saver()
if config.use_conditional_GAN:
feed_dict_init = {gan.path_placeholder: paths,
gan.semantic_map_path_placeholder: semantic_map_paths}
else:
feed_dict_init = {gan.path_placeholder: paths}
with tf.Session(config=tf.ConfigProto(allow_soft_placement=True, log_device_placement=False)) as sess:
# Initialize variables
sess.run(tf.global_variables_initializer())
sess.run(tf.local_variables_initializer())
handle = sess.run(gan.train_iterator.string_handle())
if args.restore_last and ckpt.model_checkpoint_path:
saver.restore(sess, ckpt.model_checkpoint_path)
print('Most recent {} restored.'.format(ckpt.model_checkpoint_path))
else:
if args.restore_path:
new_saver = tf.train.import_meta_graph('{}.meta'.format(args.restore_path))
new_saver.restore(sess, args.restore_path)
print('Previous checkpoint {} restored.'.format(args.restore_path))
sess.run(gan.train_iterator.initializer, feed_dict=feed_dict_init)
eval_dict = {gan.training_phase: False, gan.handle: handle}
if args.output_path is None:
output = os.path.splitext(os.path.basename(args.image_path))
save_path = os.path.join(directories.samples, '{}_compressed.pdf'.format(output[0]))
else:
save_path = args.output_path
Utils.single_plot(0, 0, sess, gan, handle, save_path, config, single_compress=True)
print('Reconstruction saved to', save_path)
return
@app.route('/output')
def done1():
return render_template('output.html');
@app.route('/contact')
def done2():
return render_template('contact.html');
@app.route('/')
def home():
return render_template('upload.html');
def allowed_file(filename):
return '.' in filename and \
filename.rsplit('.', 1)[1].lower() in ALLOWED_EXTENSIONS
class Complex:
def __init__(self,image_path,output_path,dataset,restore_path,restore_last):
self.image_path=image_path;
self.output_path=output_path;
self.dataset=dataset;
self.restore_path=restore_path
self.restore_last=restore_last
@app.route('/uploader', methods=['GET', 'POST'])
def upload_file():
if request.method == 'POST':
# check if the post request has the file part
if 'file' not in request.files:
flash('No file part')
return redirect(request.url)
file = request.files['file']
# if user does not select file, browser also
# submit an empty part without filename
if file.filename == '':
flash('No selected file')
return redirect(request.url)
if file and allowed_file(file.filename):
file_hash = hashlib.md5(file.read()).hexdigest()
print("image id: ", file_hash)
save_path1 = os.path.join(app.config['UPLOAD_FOLDER'], file_hash + '.png')
file.seek(0)
file.save(save_path1)
args=Complex(save_path1,OUTPUT_FOLDER,"cityscapes","path to model to be restored","restore last saved model")
single_compress(config_test,args);
return redirect(url_for('done1'))
if __name__ == '__main__':
app.run()
<file_sep>import cv2
import matplotlib.pyplot as plt
import glob
for name in glob.glob('train/*'):
img = cv2.imread(name, cv2.IMREAD_COLOR)
print('Original size',img.shape)
height = 512
width = 1024
dim = (width, height)
res = cv2.resize(img, dim, interpolation=cv2.INTER_LINEAR)
# Checcking the size
print("RESIZED", res.shape)
if cv2.imwrite(name[7:],res) :
print("saved "+name[7:])
| 5f67f218329b95326787d71701e0e6f8925588bc | [
"Markdown",
"Python"
] | 4 | Markdown | vishrutKulkarni1010/RVPS_Image_Compression_GAN | e331d6c3dda78f14e0cb44bbec545e3691da3189 | fb4ef297f93d1b4080e4ddadc4e53049a542118d |
refs/heads/main | <file_sep>package com.higradius;
import java.io.BufferedReader;
import java.io.IOException;
import java.sql.Connection;
import java.sql.PreparedStatement;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class EditSalesOrder
*/
@WebServlet("/EditSalesOrder")
public class EditSalesOrder extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public EditSalesOrder() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
response.getWriter().append("Served at: ").append(request.getContextPath());
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
String salesOrder = null;
try {
BufferedReader reader = request.getReader();
salesOrder = reader.readLine();
System.out.println(salesOrder);
salesOrder = salesOrder.substring(1, salesOrder.length() - 1);
String final_values[] = salesOrder.split(",");
for(int i = 0; i < final_values.length; ++i) {
final_values[i] = final_values[i].split(":")[1];
if(final_values[i].charAt(0) == '\"') {
final_values[i] = final_values[i].substring(1, final_values[i].length() - 1);
}
System.out.println(final_values[i]);
}
String salesOrderNumber = final_values[0];
String salesOrderAmount = final_values[1];
String notes = final_values[2];
Connection conn = GetConnection.connectToDB();
String sql_statement = "UPDATE invoice_details SET total_open_amount = ?, notes = ? WHERE doc_id = ?";
PreparedStatement st = conn.prepareStatement(sql_statement);
st.setString(3, salesOrderNumber);
st.setString(1, salesOrderAmount);
st.setString(2, notes.isEmpty() ? null : notes);
System.out.println(st);
st.executeUpdate();
conn.close();
}
catch(Exception e) {
}
}
}
<file_sep>import LandingPage from './LandingPage.js';
import ViewCorrespondencePage from './ViewCorrespondencePage.js';
import AddInvoicePage from './AddInvoicePage.js';
import DeleteInvoicePage from './DeleteInvoicePage.js';
import EditInvoicePage from './EditInvoicePage.js';
export {
LandingPage,
ViewCorrespondencePage,
AddInvoicePage,
DeleteInvoicePage,
EditInvoicePage,
};
<file_sep>import React from 'react';
import { makeStyles } from '@material-ui/core';
import { Select, MenuItem } from '@material-ui/core';
import { Dialog, IconButton, Button } from '@material-ui/core';
import MuiDialogTitle from '@material-ui/core/DialogTitle';
import MuiDialogContent from '@material-ui/core/DialogContent';
import MuiDialogActions from '@material-ui/core/DialogActions';
import { Table, TableContainer, TableHead, TableBody, TableRow, TableCell } from '@material-ui/core';
import { CloseIcon } from '../assets';
import jsPDF from 'jspdf';
import html2canvas from 'html2canvas';
const useStyles = makeStyles((theme) => ({
WindowHeader: {
background: '#2A3E4C',
borderRadius: '10px 10px 0px 0px',
position: 'static',
},
Title: {
color: '#FFFFFF',
font: 'Ubuntu',
fontWeight: 'normal',
},
TopRightMenu: {
position: 'fixed',
top: '80px',
right: '170px',
display: 'flex',
},
Dropdown: {
width: '180px',
color: '#FFFFFF',
background: '#283A46',
border: '1px solid #356680',
borderRadius: 10,
paddingLeft: '20px',
paddingTop: '3px',
paddingBottom: '3px',
},
ViewText: {
paddingTop: '16px',
paddingRight: '25px',
font: 'Ubuntu',
fontWeight: 'normal',
fontSize: '17px',
letterSpacing: '0.33px',
color: '#C0C6CA',
},
Body: {
fontSize: '15px',
color: '#97A1A9',
background: '#2A3E4C',
},
tableHeading: {
backgroundColor: '#273D49CC',
color: '#97A1A9',
fontSize: '13px',
borderBottom: 'none',
opacity: '100%',
},
tableBody: {
"&:nth-of-type(odd)": {
backgroundColor: '#273D49CC',
},
"&:nth-of-type(even)": {
backgroundColor: "#283A46"
},
color: '#FFFFFF',
},
tableRow: {
color: '#FFFFFF',
borderBottom: 'none',
height: '10px',
fontSize: '14px',
},
dialogPaper: {
minHeight: '80vh',
maxHeight: '80vh',
minWidth: '80vw',
maxWidth: '80vw',
background: '#000000',
},
CancelButton: {
color: '#14AFF1',
textTransform: 'none',
},
DownloadButton: {
color: '#FFF',
textTransform: 'none',
background: '#14AFF1',
borderRadius: '10px',
height: '30px',
paddingLeft: '15px',
paddingRight: '15px'
},
}));
function createData(invoiceNumber, PONumber, invoiceDate, dueDate, currency, openAmount) {
return { invoiceNumber, PONumber, invoiceDate, dueDate, currency, openAmount };
}
const rows = [
createData('73457346735', '73457346735', '23-Jan-2021', '02-Jan-2021', 'USD', '122.55K'),
createData('54723243652', '54723243652', '15-Jan-2021', '15-Jan-2021', 'USD', '1.45K'),
]
const InvoiceDetailsTable = ({ selectedInvoiceDetails }) => {
const classes = useStyles();
return (
<TableContainer>
<Table>
<TableHead>
<TableRow>
<TableCell className={classes.tableHeading}>Sales Order Number</TableCell>
<TableCell className={classes.tableHeading}>PO Number</TableCell>
<TableCell className={classes.tableHeading}>Sales Order Date</TableCell>
<TableCell className={classes.tableHeading}>Due Date</TableCell>
<TableCell className={classes.tableHeading}>Currency</TableCell>
<TableCell className={classes.tableHeading}>Open Amount($)</TableCell>
</TableRow>
</TableHead>
<TableBody component="th" scope="row">
{(selectedInvoiceDetails === undefined ? [] : selectedInvoiceDetails).map((row) => (
<TableRow className={classes.tableBody}>
<TableCell className={classes.tableRow}>{row['doc_id']}</TableCell>
<TableCell className={classes.tableRow}>{row['doc_id']}</TableCell>
<TableCell className={classes.tableRow}>{row['clear_date']}</TableCell>
<TableCell className={classes.tableRow}>{row['due_in_date']}</TableCell>
<TableCell className={classes.tableRow}>USD</TableCell>
<TableCell className={classes.tableRow}>{row['total_open_amount']}</TableCell>
</TableRow>
))}
</TableBody>
</Table>
</TableContainer>
)
}
const Template1 = ({ selectedInvoiceDetails }) => {
const classes = useStyles();
var amountToBePaid = 0;
for(let i = 0; i < selectedInvoiceDetails.length; ++i) {
amountToBePaid += selectedInvoiceDetails[i]['total_open_amount']
}
return (
<div className={classes.Body}>
Subject: <b>Sales Order Details - {"{Account Name}"}</b>
<p>
Dear Sir/Madam, <br/>
Greetings!
</p>
<p>
This is to remind you that there are one or more open invoices on your account. Please provide at your earliest convenience an update on the payment details or clarify the reason for the delay. If you have any specific issue with the invoice(s), please let us know so that we can address it to the correct Department. Please find the details of the invoices below:
</p>
<p>
Please find the details of the invoices below:
</p>
<div>
<InvoiceDetailsTable selectedInvoiceDetails={selectedInvoiceDetails}/>
</div>
<p>
Total Amount to be Paid: <b>${amountToBePaid}</b>
</p>
<p>
Incase you have already made the payment for the above items, please send us the details to ensure the payment is posted.<br/>
Let us know if we can be of any further assistance. Looking forward to hearing from you.
</p>
<p>
Kind Regards,<br/>
<b>[Sender's First Name][Sender's Last Name]</b><br/>
Phone: <b>[Sender's contact number]</b><br/>
Fax: <b>[If any]</b><br/>
Email: <b>[Sender's Email Address]</b><br/>
Company Name: <b>[Sender's Company Name]</b>
</p>
</div>
)
}
const Template2 = ({ selectedInvoiceDetails }) => {
const classes = useStyles();
return(
<div className={classes.Body}>
Subject: <b>Sales Order Details - {"{Account Name}"}</b>
<p>
Dear Sir/Madam,
</p>
<p>
Gentle reminder that you have one or more open invoices on your account. <br/>
Please get back to us with an expected date of payment. If you have any issue with the invoice(s), please let us know so that we can aderess it at the earliest.
</p>
<p>
Please find the details of the invoices below:
</p>
<div>
<InvoiceDetailsTable selectedInvoiceDetails={selectedInvoiceDetails}/>
</div>
<p>
Incase you have already made the payment for the above items, please send us the details to ensure the payment is posted.<br/>
Let us know if we can be of any further assistance. Looking forward to hearing from you.
</p>
<p>
Kind Regards,<br/>
<b>[Sender's First Name][Sender's Last Name]</b><br/>
Phone: <b>[Sender's contact number]</b><br/>
Fax: <b>[If any]</b><br/>
Email: <b>[Sender's Email Address]</b><br/>
Company Name: <b>[Sender's Company Name]</b>
</p>
</div>
)
}
const ViewCorrespondencePage = ({ open, setOpen, selectedInvoiceDetails }) => {
const classes = useStyles();
const [ maxWidth ] = React.useState('lg');
const [ fullWidth ] = React.useState(false);
const [ template, setTemplate ] = React.useState('Template 1');
const handleClose = () => {
setOpen(false);
}
const handleChange = (event) => {
setTemplate(event.target.value);
}
const handleDownload = () => {
var pdf = new jsPDF(template === 'Template 1' ? <Template1/> : <Template2/>);
// pdf.fromHTML(template === 'Template 1' ? <Template1/> : <Template2/>);
// html2canvas(template === 'Template 1' ? <Template1/> : <Template2/>)
// .then((canvas) => {
// const imgData = canvas.toDataURL('image/png');
// const pdf = new jsPDF();
// pdf.addImage(imgData, 'JPEG', 0, 0);
// pdf.save("download.pdf");
// })
pdf.save('download.pdf');
}
return (
<Dialog onClose={handleClose} open={open} maxWidth={maxWidth} fullWidth={fullWidth} classes={{ paper: classes.dialogPaper }}>
<MuiDialogTitle className={classes.WindowHeader} >
<div style={{ display: 'flex', paddingTop: '5px' }}>
<div className={classes.Title}>
View Correspondence (2)
</div>
<div className={classes.TopRightMenu} >
<div className={classes.ViewText}>
View:
</div>
<div style={{ paddingTop: '10px', paddingBottom: '10px', paddingRight: '30px' }}>
<Select
className={classes.Dropdown}
disableUnderline={true}
onChange={handleChange}
value={template}
>
<MenuItem value="Template 1">
<div>Template 1</div>
</MenuItem>
<MenuItem value="Template 2">
Template 2
</MenuItem>
</Select>
</div>
<IconButton onClick={handleClose}>
<CloseIcon/>
</IconButton>
</div>
</div>
</MuiDialogTitle>
<MuiDialogContent className={classes.Body}>
{template === 'Template 1' ?
<Template1 selectedInvoiceDetails={selectedInvoiceDetails}/> :
<Template2 selectedInvoiceDetails={selectedInvoiceDetails}/>
}
</MuiDialogContent>
<MuiDialogActions style={{ background: '#2A3E4C', borderRadius: '0px 0px 10px 10px' }}>
<div style={{ paddingRight: '20px' }}>
<Button autofocus onClick={handleClose} className={classes.CancelButton}>Cancel</Button>
</div>
<div style={{ paddingRight: '20px' }}>
<Button autofocus onClick={handleDownload} className={classes.DownloadButton}>Download</Button>
</div>
</MuiDialogActions>
</Dialog>
)
}
export default ViewCorrespondencePage;<file_sep>import React from 'react';
import axios from 'axios';
import { makeStyles } from '@material-ui/core';
import { ABCLogo, HRCLogo, SearchIcon, EditIcon } from '../assets'
import { pxToRem } from '../utils/theme';
import { Table, TableBody, TableCell, TableContainer, TableHead, TableRow, CircularProgress } from '@material-ui/core';
import { AppBar, Toolbar, Button, Input, InputAdornment, Checkbox } from '@material-ui/core';
import InfiniteScroll from 'react-infinite-scroll-component';
import { AddInvoicePage, DeleteInvoicePage, EditInvoicePage, ViewCorrespondencePage } from '../views';
const useStyles = makeStyles((theme) => ({
LandingPage: {
display: 'flex',
flexDirection: 'column',
},
Header: {
display: 'flex',
paddingBlock: '5px',
width: 1553.620,
},
ABCContainer: {
display: 'flex',
},
ABCLogo: {
top: pxToRem('20px'),
left: pxToRem('30px'),
width: pxToRem('44px'),
height: pxToRem('46px'),
paddingLeft: '5px',
opacity: 1,
fill: '#CD7925',
},
ABCText: {
font: 'Futura PT',
fontSize: '39px',
fontWeight: 'bold',
color: '#FFFFFF',
paddingLeft: '10px',
verticalAlign: 'center',
},
HRCLogo: {
position: 'absolute',
top: '0%',
textAlign: 'center',
paddingBlock: '10px',
width: window.innerWidth - 40,
},
InvoiceList: {
font: 'Ubuntu',
fontSize: '20px',
color: '#FFFFFF',
paddingTop: '10px',
paddingBottom: '20px',
paddingLeft: '20px',
width: '200px',
},
ToolBar: {
display: 'flex',
width: window.innerWidth - 40,
position: 'static',
background: '#273D49CC',
boxShadow: 'none',
borderBottom: 'none',
borderTopLeftRadius: 10,
borderTopRightRadius: 10,
},
TableBox: {
width: window.innerWidth - 40,
background: '#273D49CC',
paddinLeft: '20px',
paddingBottom: '20px',
borderBottomRightRadius: 10,
borderBottomLeftRadius: 10
},
DataTable: {
width: window.innerWidth - 80,
paddingLeft: '20px',
paddingBottom: '20px',
opacity: 1,
borderBottomLeftRadius: 10,
borderBottomRightRadius: 10,
},
tableHeading: {
backgroundColor: '#273D49CC',
color: '#97A1A9',
fontSize: '15px',
borderBottom: 'none',
opacity: '100%',
},
tableBody: {
"&:nth-of-type(odd)": {
backgroundColor: '#273D49CC',
},
"&:nth-of-type(even)": {
backgroundColor: "#283A46"
},
color: '#FFFFFF',
fontSize: '16px',
"&$selected, &$selected:hover": {
backgroundColor: '#2A5368'
},
borderRadius: 10,
},
hover: {},
selected: {},
tableRow: {
color: '#FFFFFF',
borderBottom: 'none',
height: '10px',
},
searchByInvoiceNumber: {
color: '#97A1A9',
borderBottom: 'none',
border: '1px solid #356680',
background: '#283A46',
borderRadius: 10,
paddingLeft: '10px',
paddingRight: '10px',
disableUnderline: true,
height: '45px',
borderBottom: '1px solid #356680',
width: '340px',
},
Button: {
color: '#FFFFFF',
border: '1px solid #14AFF1',
borderRadius: 10,
textTransform: 'none',
height: '45px',
padding: '15px',
},
DisabledButton: {
color: '#97A1A9',
border: '1px solid #97A1A9',
borderRadius: 10,
textTransform: 'none',
height: '45px',
padding: '15px',
},
checkbox: {
root: {
color: '#14AFF1',
'&$checked': {
color: '#14AFF1',
},
},
},
}));
const Header = (props) => {
const classes = useStyles();
return (
<div className={classes.Header}>
<div className={classes.ABCContainer}>
<div className={classes.ABCLogo}>
<ABCLogo/>
</div>
</div>
<div className={classes.HRCLogo}>
<HRCLogo/>
</div>
</div>
)
}
const DataTable = ({
data, setData,
selected, setSelected,
searchKeyword, searchResults,
searchPageCount, setSearchPageCount,
dataPageCount, setDataPageCount
}) => {
const classes = useStyles();
const [ isNext, setNext ] = React.useState(false);
const loadMoreData = () => {
if(viewSearchResults) {
setSearchPageCount(searchPageCount + 1);
if(dataPageCount !== 0) {
setDataPageCount(0);
}
}
else {
setDataPageCount(dataPageCount + 1);
if(searchPageCount !== 0) {
setSearchPageCount(0);
}
}
}
const handleSelectAllClick = event => {
if (event.target.checked) {
const newSelecteds = data.map(n => n['doc_id']);
setSelected(newSelecteds);
}
else {
setSelected([]);
}
};
const handleClick = (event, doc_id) => {
const selectedIndex = selected.indexOf(doc_id);
let newSelected = [];
if (selectedIndex === -1) {
newSelected = newSelected.concat(selected, doc_id);
} else if (selectedIndex === 0) {
newSelected = newSelected.concat(selected.slice(1));
} else if (selectedIndex === selected.length - 1) {
newSelected = newSelected.concat(selected.slice(0, -1));
} else if (selectedIndex > 0) {
newSelected = newSelected.concat(
selected.slice(0, selectedIndex),
selected.slice(selectedIndex + 1)
);
}
setSelected(newSelected);
};
React.useEffect(() => {
if(dataPageCount !== -1) {
setNext(true);
axios.get(`http://localhost:8080/1830196/SendData?page=${dataPageCount}`)
.then((response) => {
setData((prev) => [...prev, ...response.data]);
})
.catch((error) => {
console.log(error);
})
}
}, [ dataPageCount ]);
const isSelected = (doc_id) => selected.indexOf(doc_id) !== -1;
const dataLength = data === undefined ? 0 : data.length;
const selectedLength = selected === undefined ? 0 : selected.length;
const searchResultsLength = searchResults === undefined ? 0 : searchResults.length;
const viewSearchResults = searchKeyword !== '';
return (
<div style={{ paddingLeft: '20px' }}>
<div className={classes.TableBox}>
<TableContainer id="data-table" style={{ height: (window.innerHeight - 230), width: (window.innerWidth - 60), overflow: 'scroll', overflowX: 'hidden' }}>
<InfiniteScroll
scrollableTarget="data-table"
dataLength={viewSearchResults ? searchResultsLength : dataLength}
hasMore={isNext}
next={loadMoreData}
loader={
<div style={{ width: '100px', height: '100px', margin: 'auto'}}>
<CircularProgress/>
</div>
}
>
<Table className={classes.DataTable} stickyHeader aria-label="customized table">
<TableHead>
<TableRow>
<TableCell padding="checkbox">
<Checkbox
indeterminate={selectedLength > 0 && selectedLength < dataLength}
checked={dataLength > 0 && selectedLength === dataLength}
onChange={handleSelectAllClick}
inputProps={{ 'aria-label': 'select all desserts' }}
className={classes.checkbox}
disableRipple={true}
size='small'
/>
</TableCell>
<TableCell key={'name_custoemr'} className={classes.tableHeading}>Customer Name</TableCell>
<TableCell key={'cust_number'} className={classes.tableHeading}>Customer #</TableCell>
<TableCell key={'doc_id'} className={classes.tableHeading}>Sales Order #</TableCell>
<TableCell key={'total_open_amount'} className={classes.tableHeading} align="right">Sales Order Amount</TableCell>
<TableCell key={'due_in_date'} className={classes.tableHeading} align="right">Due Date</TableCell>
<TableCell key={'clear_date'} className={classes.tableHeading} align="right">Predicted Payment Date</TableCell>
<TableCell key={'predicted_aging_bucket'} className={classes.tableHeading}>Predicted Aging Bucket</TableCell>
<TableCell key={'notes'} className={classes.tableHeading}>Notes</TableCell>
</TableRow>
</TableHead>
<TableBody component="th" scope="row">
{(searchKeyword === '' ? data : searchResults).map((row) => {
const isItemSelected = isSelected(row['doc_id']);
return (
<TableRow
className={classes.tableBody}
classes={{ hover: classes.hover, selected: classes.selected }}
selected={isItemSelected}
>
<TableCell padding="checkbox">
<Checkbox
checked={isItemSelected}
onClick={(event) => handleClick(event, row['doc_id'])}
className={classes.tableRow}
className={classes.checkbox}
disableRipple={true}
size='small'
/>
</TableCell>
<TableCell className={classes.tableRow}>{row['name_customer']}</TableCell>
<TableCell className={classes.tableRow}>{row['cust_number']}</TableCell>
<TableCell className={classes.tableRow}>{row['doc_id']}</TableCell>
<TableCell className={classes.tableRow} align="right">{row['total_open_amount']}</TableCell>
<TableCell className={classes.tableRow} align="right">{row['due_in_date']}</TableCell>
<TableCell className={classes.tableRow} align="right">{row['clear_date'] === '' || row['clear_date'] === null ? '--' : row['clear_date']}</TableCell>
<TableCell className={classes.tableRow}>--</TableCell>
<TableCell className={classes.tableRow}>{row['notes'] === null ? '--' : row['notes']}</TableCell>
</TableRow>
);
})}
</TableBody>
</Table>
</InfiniteScroll>
</TableContainer>
</div>
</div>
)
}
const Bar = ({
data, setData,
selected, setSelected,
searchKeyword, setSearchKeyword,
searchResults, setSearchResults,
searchPageCount, setSearchPageCount,
setDataPageCount
}) => {
const classes = useStyles();
const [ openAddInvoice, setOpenAddInvoice ] = React.useState(false);
const [ openDeleteInvoice, setOpenDeleteInvoice ] = React.useState(false);
const [ openEditInvoice, setOpenEditInvoice ] = React.useState(false);
const [ openViewCorrespondence, setOpenViewCorrespondence ] = React.useState(false);
const [ selectedInvoiceDetails, setSelectedInvoiceDetails ] = React.useState([]);
const handleAddInvoice = () => {
setOpenAddInvoice(true);
}
const handleDeleteInvoice = () => {
setOpenDeleteInvoice(true);
}
const handleEditInvoice = () => {
setOpenEditInvoice(true);
}
const handleViewCorrespondence = () => {
setOpenViewCorrespondence(true);
}
const handleSearch = (event) => {
setSearchKeyword(event.target.value);
setSearchPageCount(0);
setSearchResults([]);
}
React.useEffect(() => {
axios.get(`http://localhost:8080/1830196/SearchSalesOrder?searchKeyword=${searchKeyword}&page=${searchPageCount}`)
.then((response) => {
setSearchResults([...searchResults, ...response.data]);
})
.catch((error) => {
console.log(error)
})
console.log(searchKeyword)
}, [ searchKeyword, searchPageCount ])
React.useEffect(() => {
setSelectedInvoiceDetails(data.filter(row => selected.indexOf(row['doc_id']) != -1));
}, [ selected ])
const isDisabledEditButton = (selected.length !== 1);
const isDisabledViewCorrespondenceButton = (selected.length === 0);
const isDisabledDeleteButton = (selected.length === 0);
const isDisabledPredictButton = (selected.length === 0);
return (
<AppBar className={classes.ToolBar}>
<Toolbar style={{ display: 'flex' }}>
<div style={{ display: 'flex' }}>
<div style={{ paddingRight: '20px', paddingTop: '10px', }}>
<Button
className={isDisabledPredictButton ? classes.DisabledButton : classes.Button}
disabled={isDisabledPredictButton}
>
Predict
</Button>
</div>
<div style={{ paddingRight: '10px', paddingTop: '10px', }}>
<Button
className={isDisabledViewCorrespondenceButton ? classes.DisabledButton : classes.Button}
onClick={handleViewCorrespondence}
disabled={isDisabledViewCorrespondenceButton}
>
View Correspondence
</Button>
<ViewCorrespondencePage
open={openViewCorrespondence} setOpen={setOpenViewCorrespondence}
selectedInvoiceDetails={selectedInvoiceDetails}
/>
</div>
</div>
<div style={{ position: 'fixed', right: 40, display: 'flex' }}>
<div style={{ paddingRight: '20px', paddingTop: '10px', }}>
<Button
className={classes.Button}
onClick={handleAddInvoice}
>
+ Add
</Button>
<AddInvoicePage
open={openAddInvoice} setOpen={setOpenAddInvoice}
setDataPageCount={setDataPageCount} setData={setData}
/>
</div>
<div style={{ paddingRight: '20px', paddingTop: '10px', }}>
<Button
className={isDisabledEditButton ? classes.DisabledButton : classes.Button}
onClick={handleEditInvoice}
disabled={isDisabledEditButton}
>
<EditIcon style={{ paddingRight: '10px' }}/>
Edit
</Button>
<EditInvoicePage
open={openEditInvoice} setOpen={setOpenEditInvoice}
selectedInvoiceDetails={selectedInvoiceDetails}
setDataPageCount={setDataPageCount} setData={setData}
/>
</div>
<div style={{ paddingRight: '20px', paddingTop: '10px', }}>
<Button
className={isDisabledDeleteButton ? classes.DisabledButton : classes.Button}
onClick={handleDeleteInvoice}
disabled={isDisabledDeleteButton}
>
— Delete
</Button>
<DeleteInvoicePage
open={openDeleteInvoice} setOpen={setOpenDeleteInvoice}
selected={selected}
setDataPageCount={setDataPageCount}
/>
</div>
<div style={{ paddingTop: '10px', }}>
<Input
className={classes.searchByInvoiceNumber}
placeholder='Search by Invoice Number'
disableUnderline={true}
value={searchKeyword}
onChange={(event) => handleSearch(event)}
endAdornment={
<InputAdornment position='end'>
<SearchIcon/>
</InputAdornment>
}
></Input>
</div>
</div>
</Toolbar>
</AppBar>
)
}
const LandingPage = () => {
const classes = useStyles();
const [ data, setData ] = React.useState([]);
const [ dataPageCount, setDataPageCount ] = React.useState(0);
const [ selected, setSelected ] = React.useState([]);
const [ searchKeyword, setSearchKeyword ] = React.useState('');
const [ searchResults, setSearchResults ] = React.useState([]);
const [ searchPageCount, setSearchPageCount ] = React.useState(0);
return (
<div className={classes.LandingPage}>
<div style={{paddingLeft: '20px'}}>
<Header/>
</div>
<div className={classes.InvoiceList}>
Sales Order List
</div>
<div className={classes.InvoiceTable}>
<div style={{ paddingLeft: '19px' }}>
<Bar
data={data} setData={setData}
selected={selected} setSelected={setSelected}
searchKeyword={searchKeyword} setSearchKeyword={setSearchKeyword}
searchResults={searchResults} setSearchResults={setSearchResults}
searchPageCount={searchPageCount} setSearchPageCount={setSearchPageCount}
setDataPageCount={setDataPageCount}
/>
</div>
<div>
<DataTable
data={data} setData={setData}
selected={selected} setSelected={setSelected}
searchKeyword={searchKeyword} searchResults={searchResults}
searchPageCount={searchPageCount} setSearchPageCount={setSearchPageCount}
dataPageCount={dataPageCount} setDataPageCount={setDataPageCount}
/>
</div>
</div>
</div>
)
}
export default LandingPage;<file_sep>import React from 'react';
import axios from 'axios';
import { makeStyles } from '@material-ui/core';
import { Dialog, IconButton, Button, Input } from '@material-ui/core';
import MuiDialogTitle from '@material-ui/core/DialogTitle';
import MuiDialogContent from '@material-ui/core/DialogContent';
import MuiDialogActions from '@material-ui/core/DialogActions';
import { CloseIcon } from '../assets';
const useStyles = makeStyles((theme) => ({
dialogPaper: {
minHeight: '60vh',
maxHeight: '60vh',
minWidth: '30vw',
maxWidth: '30vw',
background: '#000000',
},
WindowHeader: {
background: '#2A3E4C',
borderRadius: '10px 10px 0px 0px',
position: 'static',
},
Title: {
color: '#FFFFFF',
font: 'Ubuntu',
fontWeight: 'normal',
},
TopRightMenu: {
position: 'fixed',
top: '160px',
right: '570px',
},
Body: {
fontSize: '15px',
color: '#97A1A9',
background: '#2A3E4C',
paddingBlock: '40px',
},
InputBox: {
color: '#FFFFFF',
width: '240px',
border: '1px solid #356680',
background: '#283A46',
borderRadius: 10,
paddingLeft: '10px',
paddingRight: '10px'
},
ErrorInputBox: {
color: '#FFFFFF',
width: '240px',
border: '1px solid #FF5B5B',
background: '#283A46',
borderRadius: 10,
paddingLeft: '10px',
paddingRight: '10px'
},
CancelButton: {
color: '#14AFF1',
textTransform: 'none',
},
ResetButton: {
color: '#FFF',
textTransform: 'none',
border: '1px solid #14AFF1',
borderRadius: 10,
height: '30px',
},
SaveButton: {
color: '#FFF',
textTransform: 'none',
background: '#14AFF1',
borderRadius: '10px',
height: '30px',
paddingLeft: '15px',
paddingRight: '15px'
},
}))
const EditMenu = ({
invoiceAmount, notes,
newInvoiceAmount, setNewInvoiceAmount,
newNotes, setNewNotes,
setSaveButtonClicked,
isErrorNewInvoiceAmount
}) => {
const classes = useStyles();
const handleInvoiceAmount = (event) => {
setSaveButtonClicked(false);
setNewInvoiceAmount(event.target.value);
}
const handleNotes = (event) => {
setSaveButtonClicked(false);
setNewNotes(event.target.value);
}
return (
<div style={{ display: 'flex' }}>
<div style={{ paddingLeft: '10px', paddingTop: '5px', fontSize: '17px' }}>
<div style={{ paddingBottom: '45px', paddingRight: '10px', display: 'flex', width: '160px' }}>
Sales Order Amount
</div>
<div style={{ paddingBottom: '45px', display: 'flex' }}>
Notes
</div>
</div>
<div>
<div style={{ paddingBottom: '30px' }}>
<Input
className={isErrorNewInvoiceAmount ? classes.ErrorInputBox : classes.InputBox}
disableUnderline={true}
value={newInvoiceAmount}
placeholder={invoiceAmount}
onChange={(event) => handleInvoiceAmount(event)}
>
</Input>
</div>
<div style={{ paddingBottom: '30px' }}>
<Input
className={classes.InputBox}
style={{ height: '150px' }}
disableUnderline={true}
value={newNotes}
placeholder={notes}
onChange={(event) => handleNotes(event)}
>
</Input>
</div>
</div>
</div>
)
}
const EditInvoicePage = ({
open, setOpen,
selectedInvoiceDetails,
setDataPageCount, setData
}) => {
const classes = useStyles();
const [ maxWidth ] = React.useState('lg');
const [ fullWidth ] = React.useState(false);
const invoiceNumber = selectedInvoiceDetails.length === 0 ? '' : selectedInvoiceDetails[0]['doc_id']
const invoiceAmount = selectedInvoiceDetails.length === 0 ? '' : selectedInvoiceDetails[0]['total_open_amount']
const notes = selectedInvoiceDetails.length === 0 ? '' : selectedInvoiceDetails[0]['notes'];
const [ newInvoiceAmount, setNewInvoiceAmount ] = React.useState(invoiceAmount);
const [ newNotes, setNewNotes ] = React.useState(notes);
const [ saveButtonClicked, setSaveButtonClicked ] = React.useState(false);
const isErrorNewInvoiceAmount = (newInvoiceAmount === '' && saveButtonClicked);
const handleSave = () => {
if(newInvoiceAmount !== '') {
axios.post('http://localhost:8080/1830196/EditSalesOrder',
{
invoiceNumber,
newInvoiceAmount,
newNotes
})
.then((response) => {
console.log(response);
handleClose();
// setData([])
// setDataPageCount(0);
})
.catch((error) => {
console.log(error);
})
}
setSaveButtonClicked(true);
}
const handleReset = () => {
setNewInvoiceAmount(invoiceAmount);
setNewNotes(notes);
}
const handleClose = () => {
setOpen(false);
}
return (
<Dialog onCLose={handleClose} open={open} maxWidth={maxWidth} fullWidth={fullWidth} classes={{ paper: classes.dialogPaper }}>
<MuiDialogTitle className={classes.WindowHeader}>
<div style={{ display: 'flex' }}>
<div className={classes.Title}>
Edit Invoice
</div>
<div className={classes.TopRightMenu}>
<IconButton onClick={handleClose}>
<CloseIcon/>
</IconButton>
</div>
</div>
</MuiDialogTitle>
<MuiDialogContent className={classes.Body}>
<EditMenu
invoiceAmount={invoiceAmount} notes={notes}
newInvoiceAmount={newInvoiceAmount} setNewInvoiceAmount={setNewInvoiceAmount}
newNotes={newNotes} setNewNotes={setNewNotes}
saveButtonClicked={saveButtonClicked} setSaveButtonClicked={setSaveButtonClicked}
isErrorNewInvoiceAmount={isErrorNewInvoiceAmount}
/>
</MuiDialogContent>
<MuiDialogActions style={{ background: '#2A3E4C', borderRadius: '0px 0px 10px 10px' }}>
<div style={{ position: 'fixed', left: '585px', paddingBottom: '10px' }}>
<Button
autofocus
className={classes.CancelButton}
onClick={handleClose}
>
Cancel
</Button>
</div>
<div style={{ paddingRight: '20px', paddingBottom: '10px' }}>
<Button
autofocus
className={classes.ResetButton}
onClick={handleReset}>Reset</Button>
</div>
<div style={{ paddingRight: '20px', paddingBottom: '10px' }}>
<Button
autofocus
className={classes.SaveButton}
onClick={handleSave}>Save</Button>
</div>
</MuiDialogActions>
</Dialog>
);
}
export default EditInvoicePage;<file_sep>import axios from 'axios';
import { SERVER_URL,ROLL_NUMBER } from '../utils/constants';
export function serviceCall() {
return axios.post(`${SERVER_URL}`);
}
export function callDummyAPI(name) {
return axios.post(
`${SERVER_URL}${ROLL_NUMBER}/dummy.do?`,
{},
{
headers: { 'Content-Type': 'application/json' },
params: { name: name },
}
);
}
<file_sep>package com.higradius;
import java.sql.Connection;
import java.sql.DriverManager;
public class GetConnection {
// JDBC Driver Name and Database URL
static final String JDBC_DRIVER = "com.mysql.cj.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost:3306/invoices";
// Database credentials
static final String USER = "root";
static final String PASS = "<PASSWORD>";
public static Connection connectToDB() throws Exception {
// Register JDBC Driver
Class.forName(JDBC_DRIVER);
// Open a connection
Connection conn = DriverManager.getConnection(DB_URL, USER, PASS);
return conn;
}
}
<file_sep>import React from 'react';
import axios from 'axios';
import { makeStyles } from '@material-ui/core';
import { Dialog, IconButton, Button, Input } from '@material-ui/core';
import MuiDialogTitle from '@material-ui/core/DialogTitle';
import MuiDialogContent from '@material-ui/core/DialogContent';
import MuiDialogActions from '@material-ui/core/DialogActions';
import { MuiPickersUtilsProvider, KeyboardDatePicker } from '@material-ui/pickers';
import 'date-fns';
import DateFnsUtils from '@date-io/date-fns';
import { CloseIcon, MandatoryFieldsLogo } from '../assets';
import { Fragment } from 'react';
const useStyles = makeStyles((theme) => console.log(theme) || ({
dialogPaper: {
minHeight: '60vh',
maxHeight: '60vh',
minWidth: '60vw',
maxWidth: '60vw',
background: '#000000',
},
errorbox: {
minHeight: '8vh',
maxHeight: '8vh',
minWidth: '25vw',
maxWidth: '25vw',
position: 'absolute',
bottom: '10px',
left: '10px',
background: '#000000',
},
WindowHeader: {
background: '#2A3E4C',
borderRadius: '10px 10px 0px 0px',
position: 'static',
},
Title: {
color: '#FFFFFF',
font: 'Ubuntu',
fontWeight: 'normal',
},
TopRightMenu: {
position: 'fixed',
top: '160px',
right: '330px',
},
Body: {
fontSize: '15px',
color: '#97A1A9',
background: '#2A3E4C',
paddingBlock: '40px',
},
Column1: {
width: '30vw',
height: '30vh',
paddingLeft: '40px'
},
Column2: {
width: '30vw',
height: '30vh',
paddingLeft: '50px'
},
InputBox: {
color: '#FFFFFF',
width: '205px',
border: '1px solid #356680',
background: '#283A46',
borderRadius: 10,
paddingLeft: '10px',
paddingRight: '10px',
},
ErrorInputBox: {
color: '#FFFFFF',
width: '205px',
border: '1px solid #FF5B5B',
background: '#283A46',
borderRadius: 10,
paddingLeft: '10px',
paddingRight: '10px',
},
CancelButton: {
color: '#14AFF1',
textTransform: 'none',
},
ClearButton: {
color: '#FFF',
textTransform: 'none',
border: '1px solid #14AFF1',
borderRadius: 10,
height: '30px',
},
AddButton: {
color: '#FFF',
textTransform: 'none',
background: '#14AFF1',
borderRadius: '10px',
height: '30px',
paddingLeft: '15px',
paddingRight: '15px'
},
}))
const MandatoryFieldsPopUp = ({ open, setOpen }) => {
const classes = useStyles();
const [ maxWidth ] = React.useState('lg');
const [ fullWidth ] = React.useState(false);
const handleClose = () => {
setOpen(false);
}
return (
<Dialog
onCLose={handleClose}
open={open}
maxWidth={maxWidth}
fullWidth={fullWidth}
classes={{ paper: classes.errorbox }}
>
<MuiDialogContent
className={classes.Body}
style={{
overflow: 'hidden',
borderLeft: '5px solid #FF5B5B',
borderRadius: 10,
color: '#FFFFFF',
display: 'flex',
}}
>
<div style={{ paddingRight: '10px' }}>
<MandatoryFieldsLogo/>
</div>
<div>
Mandatory fields can't be empty
</div>
<div style={{ position: 'absolute', right: '10px', top: '8px' }}>
<IconButton onClick={handleClose}>
<CloseIcon/>
</IconButton>
</div>
</MuiDialogContent>
</Dialog>
);
}
const DatePicker = ({ dueDate, setDueDate, setAddButtonClicked, isErrorDueDate }) => {
const classes = useStyles();
const handleDueDate = (date) => {
setAddButtonClicked(false);
setDueDate(date);
}
return (
<MuiPickersUtilsProvider utils={DateFnsUtils}>
<KeyboardDatePicker
className={isErrorDueDate ? classes.ErrorInputBox : classes.InputBox}
disableToolbar
variant="inline"
error={isErrorDueDate}
onChange={handleDueDate}
format='dd/MM/yyyy'
invalidDateMessage=''
value={dueDate}
InputProps={{
disableUnderline: true,
color: 'primary',
}}
/>
</MuiPickersUtilsProvider>
)
}
const AddMenu = ({
customerName, setCustomerName,
customerNumber, setCustomerNumber,
invoiceNumber, setInvoiceNumber,
invoiceAmount, setInvoiceAmount,
dueDate, setDueDate,
notes, setNotes,
addButtonClicked, setAddButtonClicked,
}) => {
const classes = useStyles();
const handleCustomerName = (event) => {
setAddButtonClicked(false);
setCustomerName(event.target.value);
}
const handleCustomerNumber = (event) => {
setAddButtonClicked(false);
setCustomerNumber(event.target.value);
}
const handleInvoiceNumber = (event) => {
setAddButtonClicked(false);
setInvoiceNumber(event.target.value);
}
const handleInvoiceAmount = (event) => {
setAddButtonClicked(false);
setInvoiceAmount(event.target.value);
}
const handleNotes = (event) => {
setAddButtonClicked(false);
setNotes(event.target.value);
}
const isErrorCustomerName = (customerName === '' && addButtonClicked);
const isErrorCustomerNumber = (customerNumber === '' && addButtonClicked);
const isErrorInvoiceNumber = (invoiceNumber === '' && addButtonClicked);
const isErrorInvoiceAmount = (invoiceAmount === '' && addButtonClicked);
const isErrorDueDate = (dueDate === '' && addButtonClicked);
return (
<div style={{ display: 'flex' }}>
<div className={classes.Column1}>
<div style={{ display: 'flex' }}>
<div style={{ display: 'flex', flexDirection: 'column', paddingRight: '30px', paddingTop: '5px', fontSize: '17px' }}>
<div style={{ paddingBottom: '45px', display: 'flex' }}>
Customer Name<div style={{ color: 'red', paddingLeft: '5px' }}>*</div>
</div>
<div style={{ paddingBottom: '45px', display: 'flex' }}>
Customer No.<div style={{ color: 'red', paddingLeft: '5px' }}>*</div>
</div>
<div style={{ paddingBottom: '45px', display: 'flex' }}>
Sales Order No.<div style={{ color: 'red', paddingLeft: '5px' }}>*</div>
</div>
<div style={{ paddingBottom: '45px', display: 'flex' }}>
Sales Order Amount<div style={{ color: 'red', paddingLeft: '5px' }}>*</div>
</div>
</div>
<div>
<div style={{ paddingBottom: '30px' }}>
<Input
className={isErrorCustomerName ? classes.ErrorInputBox : classes.InputBox}
disableUnderline={true}
required={true}
value={customerName}
onChange={(event) => handleCustomerName(event)}
error={isErrorCustomerName}
>
</Input>
</div>
<div style={{ paddingBottom: '30px' }}>
<Input
className={isErrorCustomerNumber ? classes.ErrorInputBox : classes.InputBox}
disableUnderline={true}
required={true}
value={customerNumber}
onChange={(event) => handleCustomerNumber(event)}
error={isErrorCustomerNumber}
>
</Input>
</div>
<div style={{ paddingBottom: '30px' }}>
<Input
className={isErrorInvoiceNumber ? classes.ErrorInputBox : classes.InputBox}
disableUnderline={true}
required={true}
value={invoiceNumber}
onChange={(event) => handleInvoiceNumber(event)}
error={isErrorInvoiceNumber}
>
</Input>
</div>
<div>
<Input
className={isErrorInvoiceAmount ? classes.ErrorInputBox : classes.InputBox}
disableUnderline={true}
required={true}
value={invoiceAmount}
onChange={(event) => handleInvoiceAmount(event)}
error={isErrorInvoiceAmount}
>
</Input>
</div>
</div>
</div>
</div>
<div className={classes.Column2}>
<div style={{ display: 'flex' }}>
<div style={{ display: 'flex', flexDirection: 'column', paddingRight: '30px', paddingTop: '5px', fontSize: '17px' }}>
<div style={{ paddingBottom: '45px', display: 'flex' }}>
Due Date <div style={{ color: 'red', paddingLeft: '5px' }}>*</div>
</div>
<div style={{ paddingBottom: '45px', display: 'flex' }}>
Notes
</div>
</div>
<div>
<div style={{ paddingBottom: '30px' }}>
<DatePicker
dueDate={dueDate}
setDueDate={setDueDate}
addButtonClicked={addButtonClicked}
setAddButtonClicked={setAddButtonClicked}
isErrorDueDate={isErrorDueDate}
/>
</div>
<div style={{ paddingBottom: '30px' }}>
<Input
className={classes.InputBox}
style={{ height: '170px', width: '230px' }}
disableUnderline={true}
value={notes}
onChange={(event) => handleNotes(event)}
>
</Input>
</div>
</div>
</div>
</div>
</div>
)
}
const AddInvoicePage = ({ open, setOpen, setDataPageCount, setData }) => {
const classes = useStyles();
const [ maxWidth ] = React.useState('lg');
const [ fullWidth ] = React.useState(false);
const [ customerName, setCustomerName ] = React.useState('');
const [ customerNumber, setCustomerNumber ] = React.useState('');
const [ invoiceNumber, setInvoiceNumber ] = React.useState('');
const [ invoiceAmount, setInvoiceAmount ] = React.useState('');
const [ dueDate, setDueDate ] = React.useState('');
const [ notes, setNotes ] = React.useState('');
const [ addButtonClicked, setAddButtonClicked ] = React.useState(false);
const [ openMandatoryFieldsPopUp, setOpenMandatoryFieldsPopUp ] = React.useState(false);
const handleAddButton = () => {
setAddButtonClicked(true);
if(
customerName !== '' &&
customerNumber !== '' &&
invoiceNumber !== '' &&
invoiceAmount !== '' &&
dueDate !== ''
) {
axios.post(
'http://localhost:8080/1830196/AddInvoice',
{
customerName,
customerNumber,
invoiceNumber,
invoiceAmount,
dueDate,
notes
}
)
.then((response) => {
console.log(response);
// setData([])
// setDataPageCount(0);
})
.catch((error) => {
console.log(error);
});
handleClose();
}
else {
setOpenMandatoryFieldsPopUp(true);
}
}
const handleClearButton = () => {
setAddButtonClicked(false);
setCustomerName('');
setCustomerNumber('');
setInvoiceNumber('');
setInvoiceAmount('');
setDueDate('');
setNotes('');
}
const handleClose = () => {
handleClearButton();
setOpen(false);
}
return (
<Fragment>
<Fragment>
<Dialog onCLose={handleClose} open={open} maxWidth={maxWidth} fullWidth={fullWidth} classes={{ paper: classes.dialogPaper }}>
<MuiDialogTitle className={classes.WindowHeader} >
<div style={{ display: 'flex' }}>
<div className={classes.Title}>
Add Invoice
</div>
<div className={classes.TopRightMenu}>
<IconButton onClick={handleClose}>
<CloseIcon/>
</IconButton>
</div>
</div>
</MuiDialogTitle>
<MuiDialogContent className={classes.Body}>
<AddMenu
customerName={customerName} setCustomerName={setCustomerName}
customerNumber={customerNumber} setCustomerNumber={setCustomerNumber}
invoiceNumber={invoiceNumber} setInvoiceNumber={setInvoiceNumber}
invoiceAmount={invoiceAmount} setInvoiceAmount={setInvoiceAmount}
dueDate={dueDate} setDueDate={setDueDate}
notes={notes} setNotes={setNotes}
addButtonClicked={addButtonClicked} setAddButtonClicked={setAddButtonClicked}
/>
</MuiDialogContent>
<MuiDialogActions style={{ background: '#2A3E4C', borderRadius: '0px 0px 10px 10px' }}>
<div onClick={handleClose} style={{ position: 'fixed', left: '350px', paddingBottom: '10px' }}>
<Button autofocus className={classes.CancelButton}>Cancel</Button>
</div>
<div style={{ paddingRight: '20px', paddingBottom: '10px' }}>
<Button autofocus className={classes.ClearButton} onClick={handleClearButton}>Clear</Button>
</div>
<div style={{ paddingRight: '20px', paddingBottom: '10px' }}>
<Button autofocus className={classes.AddButton} onClick={handleAddButton}>Add</Button>
</div>
</MuiDialogActions>
</Dialog>
</Fragment>
<Fragment>
<MandatoryFieldsPopUp
open={openMandatoryFieldsPopUp}
setOpen={setOpenMandatoryFieldsPopUp}
/>
</Fragment>
</Fragment>
)
}
export default AddInvoicePage; | 1aeb50c24a4b1ab004f48759aecd6390a87700b6 | [
"JavaScript",
"Java"
] | 8 | Java | Vashisht8953/HighRadius-Invoice-Project | eab6013e20972ea17446e97e9da5e839c1e322cf | 4893abece0b75f136c610ae6c828716b5995fd8b |
refs/heads/master | <file_sep>
declare numero=0
read -p "Ingrese un numero: " numero
if [ $numero != 10 ]; then
echo "El numero ingresado no es 10"
fi
exit 0
<file_sep>CREATE TABLE customer.person(
cuit text PRIMARY KEY,
iibb text UNIQUE
);
<file_sep>CREATE TABLE provider.provider (
name text NOT NULL,
cuit text PRIMARY KEY,
iibb text UNIQUE,
address text NOT NULL
);
INSERT INTO provider.provider VALUES
('<NAME>', '20-23444555-2', '34222123', 'Salta 123'),
('<NAME>', '20-20222333-4', '12342178', 'San Martín 342'),
('<NAME>', '22-33444555-2', '45222333', 'Buenos Aires 1324'),
('<NAME>', '22-34323456-3', '892235678', 'Rivadavia 1333');
<file_sep>#!/bin/bash
declare townName=""
read -p"Ingrese el nombre de su barrio: " townName
declare postalDirection=""
read -p"Ingrese la direccion de la comisaria: " postalDirection
declare crossStreet1=""
read -p"Ingrese la primer entre calle: " crossStreet1
declare crossStreet2=""
read -p"Ingrese la segunda entre calle: " crossStreet2
echo "La comisaria de $townName se encuentra en la calle $postalDirection entre $crossStreet1 y $crossStreet2"
exit 0
<file_sep>
print("Bienvenidos al curso de python")
print("Mi nombre es martin")
<file_sep>
declare num1=0
read -p "Ingrese el primer numero: " num1
declare num2=0
read -p "Ingrese el segundo numero: " num2
declare num3=0
read -p "Ingrese el tercer numero: " num3
declare num4=0
read -p "Ingrese el cuarto numero: " num4
if [[ $num1 = $num2 || $num3 = $num4 ]]
then
echo "El primer y el segundo numero son iguales"
echo "o el tercer y el cuarto numero son iguales"
fi
<file_sep>
declare max=0
declare num=0
max=$num
echo "ingresar numeros enteros positivos de forma indefinida hasta que se ingrese 0 y mostrar el mayor"
while :
do
read -p "Ingrese numeros: " num
if (( $num > $max ));
then
max=$num
fi
(( $num > 0 ))||break
done
echo "El mayor es: $max"
exit 0
<file_sep>
num1=int(input("Ingrese primer numero: "))
num2=int(input("Ingrese segundo numero: "))
suma= num1+num2
print("El resultado de la suma es: ",suma)
<file_sep>
num1=int(input("Ingrese el primer numero: "))
num2=int(input("Ingrese el segundo numero: "))
num3=int(input("Ingrese el tercer numero: "))
num4=int(input("Ingrese el cuarto numero: "))
if num1 == num2 or num3 == num4:
print("El primer numero es igual al segundo o el tercero es igual al cuarto numero")
<file_sep>SELECT
property_id,
surface
FROM
property
WHERE
surface=(SELECT MIN(surface) FROM property)
ORDER BY 1 ASC;<file_sep>.PHONY: clean com_diary_controller/com_diary_controller.sql
PSQL = /usr/bin/psql
all: create
create: mtorrents.sql
$(PSQL) -c "DROP DATABASE IF EXISTS mtorrents;" postgres
$(PSQL) -c "CREATE DATABASE mtorrents;" postgres
$(PSQL) $(PGDATABASE) < mtorrents.sql
mtorrents.sql: \
com_diary_controller/com_diary_controller.sql
touch mtorrents.sql
cat com_diary_controller/com_diary_controller_adt.sql >> mtorrents.sql
com_diary_controller/com_diary_controller.sql:
$(MAKE) -C com_diary_controller
clean:
$(PSQL) -c "DROP DATABASE IF EXISTS mtorrents;" postgres
$(RM) mtorrents.sql
$(MAKE) -C com_diary_controller clean
<file_sep>vector1=[]
vector2=[]
print("Ingrese 5 numeros en un vector")
for i in range(1,6):
vector1.append(int(input()))
for i in range(1,12):
vector2[i]=i*2
print("",vector2[i])
<file_sep>CREATE TABLE customer.customer (
surname text NOT NULL,
name text NOT NULL,
cuit text PRIMARY KEY,
address text NOT NULL
);
INSERT INTO customer.customer VALUES
('Perez', 'Juan', '20-23444555-2', 'Salta 123'),
('Garcia', 'Pedro', '20-25666777-2', 'San Martín 342'),
('Vazquez', 'Ramon', '20-20222333-4', 'Junín 5643'),
('Rebolledo', 'Jaime', '22-18456282-1', 'Moreno 2464');
<file_sep>
print("Numeros pares del 1 al 100: ")
for i in range(1,100):
if(i%2==0):
print("",i)
<file_sep>
i=1
sum=0
for i in range(1,101):
sum=sum+i
print("La suma de los numeros del 1 al 100 es: ",sum)
<file_sep>CREATE TABLE person(
dni integer PRIMARY KEY,
name text NOT NULL,
surname text NOT NULL,
nationality text NOT NULL,
birthday date NOT NULL,
sex text CHECK(sex='Femenino' OR sex='Masculino')
);
<file_sep>
declare carac
carac=""
read -p "Ingrese un caracter cualquiera(numero,consonante,vocal): " carac
if [ $carac = "a" ] || [ $carac = "e" ] || [ $carac = "i" ] || [ $carac = "o" ] || [ $carac = "u" ]
then
echo "El caracter ingresado es una vocal"
elif [ $carac = "b" ] || [ $carac = "c" ] || [ $carac = "d" ] || [ $carac=="f" ] || [ $carac = "g" ]
then
echo "El caracter ingresado es una consonante"
elif [ $carac = "0" ] || [ $carac = "1" ] || [ $carac = "2" ] || [ $carac=="3" ] || [ $carac = "4" ] || [ $carac = "5" ] || [ $carac = "6" ] || [ $carac = "7" ] || [ $carac = "8" ] || [ $carac = "9" ]
then
echo "El caracter ingresado es un numero"
else
echo "El caracter ingresado no es valido"
fi
exit 0
<file_sep>SELECT
name,
cuit
FROM
provider.provider
EXCEPT
SELECT
concat(name,' ',surname) "Nombre",
cuit "CUIT"
FROM
customer.customer;
<file_sep>length=6
nlength=length-1
i=1
j=1
for i in range(length):
for j in range(i):
print("X",end="")
print("")
for i in range(1,length):
for j in range(i,nlength):
print("X",end="")
print("")
<file_sep>.phony: clean
JC = javac
JFLAGS = -cp
CLASSPATH = .
all: jdbcExample.class
jdbcExample.class: jdbcExample.java
$(JC) $(JFLAGS) $(CLASSPATH) jdbcExample.java
clean:
$(RM) jdbcExample.class
<file_sep>declare -a array=()
for((i=2;i<=99;i++)){
if ((i % 2 != 0))
then
array[i]=$i
fi
}
echo "Numeros impares entre 1 y 100: "
for((i=2;i<=99;i++)){
if ((i % 2 != 0))
then
echo ${array[i]}
fi
}
<file_sep>CREATE TABLE course (
course_id text PRIMARY KEY,
name text UNIQUE,
description text NOT NULL,
price double precision NOT NULL CHECK(price >= 0)
);
CREATE TABLE person (
dni integer PRIMARY KEY NOT NULL,
name text NOT NULL,
surname text NOT NULL,
birthday timestamp with time zone NOT NULL
);
CREATE TABLE contact_information (
dni integer NOT NULL REFERENCES person(dni),
postal_address text NOT NULL,
phone_number text,
email text
);
CREATE TABLE student (
dni integer UNIQUE REFERENCES person(dni),
student_id text PRIMARY KEY,
inscription_date timestamp with time zone NOT NULL
);
CREATE TABLE is_student_of (
student_id text NOT NULL REFERENCES student(student_id),
course_id text NOT NULL REFERENCES course(course_id),
PRIMARY KEY (student_id, course_id)
);
CREATE TABLE professor (
dni integer UNIQUE REFERENCES person(dni),
cuil text PRIMARY KEY,
salary double precision NOT NULL CHECK (salary > 0),
signing_in_date timestamp with time zone NOT NULL
);
CREATE TABLE is_proffesor_of (
cuil text NOT NULL REFERENCES professor(cuil),
course_id text NOT NULL REFERENCES course(course_id),
PRIMARY KEY (cuil, course_id)
);
<file_sep>SELECT
b.seller_name,
b.seller_surname,
c.region_name,
count(operation)
FROM
property a
NATURAL INNER JOIN seller b
NATURAL INNER JOIN region c
WHERE
a.selling_timestamp IS NOT NULL
and a.operation='Venta'
AND c.region_name='Barcelona'
GROUP BY 1,2,3
ORDER BY 4 DESC
LIMIT 1;<file_sep>
num1=int(input("Ingrese primer numero: "))
num2=int(input("Ingrese segundo numero: "))
resta=num1-num2
print("El resultado de la resta es: ",resta)
<file_sep>#!/bin/bash
declare num1=0
declare num2=0
declare num3=0
declare num4=0
declare num5=0
echo "Ingrese 5 numeros: "
read -p "" num1
read -p "" num2
read -p "" num3
read -p "" num4
read -p "" num5
echo ""
echo "Numeros en ordenados forma descendente $num5 $num4 $num3 $num2 $num1"
exit 0
<file_sep>SELECT
count(property_id)
FROM
property;<file_sep>CREATE TABLE contact_information(
person integer NOT NULL PRIMARY KEY REFERENCES person(dni),
mobile_phone text NOT NULL,
phone text NOT NULL,
email text NOT NULL,
address text NOT NULL
);
<file_sep>
array=[]
for i in range(2,100):
if i % 2 != 0:
array.append(i)
print("Numeros impares entre 1 y 100: ")
for i in array:
if i % 2 != 0:
print(i)
<file_sep>SELECT
b.seller_surname,
b.seller_name,
b.seller_dni,
count(1) "Propiedades Vendidas"
FROM
seller b
NATURAL INNER JOIN property a
WHERE
a.selling_timestamp IS NOT NULL
GROUP BY 1,2,3
ORDER BY 4 ASC
LIMIT 1;<file_sep>SELECT
concat(name,' ',surname) "Nombre",
cuit "CUIT"
FROM
customer.customer
INTERSECT
SELECT
name,
cuit
FROM
provider.provider;
<file_sep>1) Añadir índices a la base de datos inmobiliaria según su criterio. Justificar.
2) Crear la Base de Datos banco del modelo XML de banco.xml
<file_sep>CREATE TYPE account_type AS ENUM('Caja de Ahorro', 'Cuenta Corriente');
CREATE SCHEMA customer;
CREATE SCHEMA accounting;
CREATE TABLE customer.person(
cuit text PRIMARY KEY,
iibb text UNIQUE
);
CREATE TABLE customer.contact_information(
contact_id serial PRIMARY KEY,
address text,
phone text,
email text,
cuit text REFERENCES customer.person(cuit) ON DELETE CASCADE
);
CREATE TABLE customer.physical_person(
dni text UNIQUE NOT NULL,
surname text NOT NULL,
name text NOT NULL,
birthday timestamptz NOT NULL,
cuit text PRIMARY KEY REFERENCES customer.person(cuit)
);
CREATE TABLE customer.legal_person(
cuit text PRIMARY KEY REFERENCES customer.person(cuit),
legal_name text NOT NULL,
fantasy_name text,
constitution_date timestamptz NOT NULL
);
CREATE TABLE accounting.account(
account_id serial PRIMARY KEY,
owner_cuit text REFERENCES customer.person(cuit) NOT NULL,
opening_date timestamptz NOT NULL DEFAULT now(),
account_type account_type NOT NULL DEFAULT 'Caja de Ahorro',
authorized_uncovered_balance double precision DEFAULT 0 CHECK(authorized_uncovered_balance <= 0) NOT NULL,
balance double precision DEFAULT 0 CHECK(balance >= authorized_uncovered_balance) NOT NULL
);
CREATE TABLE accounting.movement(
movement_id serial PRIMARY KEY,
movement_timestamp timestamptz DEFAULT now() NOT NULL,
movement_description text NOT NULL,
account_id integer REFERENCES accounting.account(account_id) NOT NULL,
quantity double precision CHECK(quantity != 0) NOT NULL
);
<file_sep>
carac=input("Ingrese un caracter cualquiera(numero,consonante,vocal): ")
if carac=='a' or carac=='e' or carac=='i' or carac=='o' or carac=='u':
print("El caracter ingresado es una vocal")
elif carac=='b' or carac=='c' or carac=='d' or carac=='f' or carac=='g' or carac=='h' or carac=='j' or carac=='k' or carac=='l' or carac=='m':
print("El caracter ingresado es una consonante")
elif carac=='0' or carac=='1' or carac=='2' or carac=='3' or carac=='4' or carac=='5' or carac=='6' or carac=='7' or carac=='8' or carac=='9':
print("El caracter ingresado es un numero")
else:
print("El caracter ingresado no es valido")
<file_sep>townName=input("Ingrese el nombre de su barrio: ")
postalDirection=input("Ingrese la calle de la comisaria mas cercana: ")
crossStreet1=input("Ingrese la primer entre calle: ")
crossStreet2=input("Ingrese la segunda entre calle: ")
print("\nLa comisaria de",townName,"se encuentra en la calle",postalDirection,"entre",crossStreet1,'y',crossStreet2)
<file_sep>
genero=input("Ingrese su genero: ")
if genero=="Femenino" or genero=="Masculino":
if genero=="Femenino":
print("El genero ingresado es femenino")
else:
print("El genero ingresado no es valido")
<file_sep>declare num1
num1=0
read -p "Ingresa el primer numero: " num1
declare num2
num2=0
read -p "Ingresa el segundo numero: " num2
declare num3
num3=0
read -p "Ingresa el tercer numero: " num3
if (( $num1<$num2 && $num1<$num3 ))
then
if (( $num2<$num3 ))
then
echo "$num1 $num2 $num3"
else
echo "$num1 $num3 $num2"
fi
else
if (( $num2<$num3 && $num2<$num1 ))
then
if (( $num3<$num1 ))
then
echo "$num2 $num3 $num1"
else
echo "$num2 $num1 $num3"
fi
else
if (( $num3<$num1 && $num3<$num2 ))
then
if (( $num1<$num2 ))
then
echo "$num3 $num1 $num2"
else
echo "$num3 $num2 $num1"
fi
fi
fi
fi
exit 0
<file_sep>DROP VIEW vw_property;
CREATE VIEW vw_property AS
SELECT
a.property_id "ID PROPIEDAD",
a.price "PRECIO",
concat(b.seller_surname,' ',b.seller_name) "VENDEDOR",
c.region_name "REGION"
FROM
property a
NATURAL INNER JOIN seller b
NATURAL INNER JOIN region c
ORDER BY 2 ASC;
SELECT * FROM vw_property;<file_sep>declare i
i=1
declare j
j=1
declare num
read -p"Ingrese un numero: " num
for((i=num;i<=num;i++)) {
for((j=1;j<=10;j++)) {
tabla=$((i*j))
echo "$i * $j = $tabla"
}
}
exit 0
<file_sep>CREATE SCHEMA customer;
CREATE SCHEMA provider;
CREATE TABLE customer.customer (
surname text NOT NULL,
name text NOT NULL,
cuit text PRIMARY KEY,
address text NOT NULL
);
INSERT INTO customer.customer VALUES
('Perez', 'Juan', '20-23444555-2', 'Salta 123'),
('Garcia', 'Pedro', '20-25666777-2', 'San Martín 342'),
('Vazquez', 'Ramon', '20-20222333-4', 'Junín 5643'),
('Rebolledo', 'Jaime', '22-18456282-1', 'Moreno 2464');
CREATE TABLE provider.provider (
name text NOT NULL,
cuit text PRIMARY KEY,
iibb text UNIQUE,
address text NOT NULL
);
INSERT INTO provider.provider VALUES
('<NAME>', '20-23444555-2', '34222123', 'Salta 123'),
('<NAME>', '20-20222333-4', '12342178', 'San Martín 342'),
('<NAME>', '22-33444555-2', '45222333', 'Buenos Aires 1324'),
('<NAME>', '22-34323456-3', '892235678', 'Rivadavia 1333');
<file_sep>CREATE TABLE b.products(
name text NOT NULL,
description text NOT NULL,
price integer NOT NULL
);
CREATE TABLE b.log(
id serial NOT NULL,
timestamptz timestamptz DEFAULT now(),
detail text
);<file_sep>CREATE TABLE customer.contact_information(
contact_id serial PRIMARY KEY,
address text,
phone text,
email text,
cuit text REFERENCES customer.person(cuit) ON DELETE CASCADE
);
<file_sep>SELECT
property_id,
surface
FROM
property
WHERE
surface=(SELECT MAX(surface) FROM property)
ORDER BY 1 ASC;
<file_sep>
numero=int(input("Ingrese un numero: "))
if numero % 2 == 0:
print("",numero,"es multiplo de 2")
else:
print("",numero,"no es multiplo de 2")
<file_sep>
num1=int(input("Ingrese 1 numero: "))
num2=int(input("Ingrese 2 numero: "))
num3=int(input("Ingrese 3 numero: "))
if num1<num2 and num1<num3:
if num2<num3:
print("",num1,"",num2,"",num3)
else:
print("",num1,"",num3,"",num2)
else:
if num2<num3 and num2<num1:
if num3<num1:
print("",num2,"",num3,"",num1)
else:
print("",num2,"",num1,"",num3)
else:
if num3<num1 and num3<num2:
if num1<num2:
print("",num3,"",num1,"",num2)
else:
print("",num3,"",num2,"",num1)
<file_sep>CREATE INDEX id_propiedad ON property(property_type_id);
CREATE INDEX id_region ON property(region_id);
CREATE INDEX dni_vendedor ON property(seller_dni);
//Justificaciòn: Los 3 atributos son claves externas
<file_sep>CREATE TABLE customer.legal_person(
cuit text PRIMARY KEY REFERENCES customer.person(cuit),
legal_name text NOT NULL,
fantasy_name text,
constitution_date timestamptz NOT NULL
);
<file_sep># FIXME: iterar por las dependencias en el objetivo com_diary_controller
all: com_diary_controller_adt.sql
com_diary_controller_adt.sql: \
person_adt.sql \
contact_information_adt.sql
touch com_diary_controller.sql
# ADT
cat person_adt.sql >> com_diary_controller_adt.sql
cat contact_information_adt.sql >> com_diary_controller_adt.sql
# MTD
cat person_mtd.sql >> com_diary_controller_adt.sql
cat contact_information_mtd.sql >> com_diary_controller_adt.sql
clean:
$(RM) com_diary_controller_adt.sql
<file_sep>
declare num1=0
declare num2=0
declare resta=0
echo "Ingrese 2 numeros: "
read -p "" num1
read -p "" num2
resta=$(($num1-$num2))
echo "El resultado de la resta es: $resta"
exit 0
<file_sep>
declare numero=0
read -p "Ingrese un numero: " numero
if (( $numero % 2 == 0 ))
then
echo "$numero es multiplo de 2"
else
echo "$numero no es multiplo de 2"
fi
exit 0
<file_sep>
for i in range(2,100):
if(i%2!=0):
print("",i)
<file_sep>
romano=str(input("Ingrese un numero romano: "))
if romano=="I":
print("Vale 1")
elif romano=="V":
print("Vale 5")
elif romano=="X":
print("Vale 10")
elif romano=="L":
print("Vale 50")
elif romano=="C":
print("Vale 100")
elif romano=="D":
print("Vale 500")
elif romano=="M":
print("Vale 1000")
else:
print("No es un numero romano valido")
<file_sep>CREATE TYPE account_type AS ENUM('Caja de Ahorro', 'Cuenta Corriente');
CREATE SCHEMA customer;
CREATE SCHEMA accounting;
<file_sep>
print("ingresar numeros enteros positivos de forma indefinida hasta que se ingrese 0 y mostrar el mayor")
num=0
maxn=0
maxn=num
while True:
num=int(input("Ingrese numeros: "))
if num>maxn:
maxn=num
if num == 0:
break
print("El mayor es: ",maxn)
<file_sep>declare num1=0
declare num2=0
declare repeat="n"
declare sum=0
echo "Ingrese 2 numeros para sumarlos y repetir esto hasta que el usuario lo disponga: "
while :
do
read -p "Ingrese un numero: " num1
read -p "Ingrese otro numero: " num2
sum=$(( num1 + num2 ))
echo "El resultado de la suma es: $sum"
read -p "Desea continuar (s/n): " repeat
[[ $repeat == "s" ]]||break
done
exit 0
<file_sep>numero=0
print("Ingrese numeros hasta que alguno sea mayor a 100")
while True:
numero=int(input("Ingrese numero: "))
if numero > 100:
break
<file_sep>declare i
declare sum
i=1
sum=0
for((i=1;i<=100;i++)){
sum=$((sum + i))
}
echo "La suma de numeros del 1 al 100 es: $sum"
exit 0
<file_sep>
base=int(input("Ingrese la base de un rectangulo: "))
height=int(input("Ingrese la altura de un rectangulo: "))
if base>0 and height>0:
for i in range(1,height):
for j in range(1,base):
print("X",end="")
print("")
print("\n")
else:
print("La base y la altura deben ser mayor a 0")
<file_sep>DROP VIEW vw_region_report;
CREATE VIEW vw_region_report AS
SELECT
concat(b.seller_surname,' ',b.seller_name) "Vendedor",
c.region_name "Region",
count(a.property_id)"Cantidad de Propiedades",
round(avg(a.price::numeric)) "Precio Promedio"
FROM
property a
NATURAL INNER JOIN seller b
NATURAL INNER JOIN region c
GROUP BY 1,2;
SELECT * FROM vw_region_report;
<file_sep>.phony: clean
CLIENT = psql
FLAGS = -c
all: deploy
deploy: banco.sql \
functions.sql \
getters.sql
$(CLIENT) $(FLAGS) "DROP DATABASE IF EXISTS banco" postgres
$(CLIENT) $(FLAGS) "CREATE DATABASE banco" postgres
$(CLIENT) banco < banco.sql
$(CLIENT) banco < functions.sql
$(CLIENT) banco < getters.sql
banco.sql: \
configuration.sql \
customer/person_adt.sql \
customer/contact_information_adt.sql \
customer/physical_person_adt.sql \
customer/legal_person_adt.sql \
accounting/account_adt.sql \
accounting/movement_adt.sql
touch banco.sql
cat configuration.sql >> banco.sql
cat customer/person_adt.sql >> banco.sql
cat customer/contact_information_adt.sql >> banco.sql
cat customer/physical_person_adt.sql >> banco.sql
cat customer/legal_person_adt.sql >> banco.sql
cat accounting/account_adt.sql >> banco.sql
cat accounting/movement_adt.sql >> banco.sql
functions.sql: \
functions/f1_physical_person_adt.sql \
functions/f2_physical_person_adt.sql \
functions/f3_customer_person_adt.sql \
functions/f4_customer_person_adt.sql \
functions/f5_customer_physical_person_adt.sql \
functions/f6_customer_contact_information_adt.sql \
functions/f7_customer_legal_person_adt.sql \
functions/f8_accounting_account_adt.sql
touch functions.sql
cat functions/f1_physical_person_adt.sql >> functions.sql
cat functions/f2_physical_person_adt.sql >> functions.sql
cat functions/f3_customer_person_adt.sql >> functions.sql
cat functions/f4_customer_person_adt.sql >> functions.sql
cat functions/f5_customer_physical_person_adt.sql >> functions.sql
cat functions/f6_customer_contact_information_adt.sql >> functions.sql
cat functions/f7_customer_legal_person_adt.sql >> functions.sql
cat functions/f8_accounting_account_adt.sql >> functions.sql
getters.sql: \
getters/g1_customer_physical_person_identify_by_dni_adt.sql \
getters/g2_customer_physical_person_identify_by_cuit_adt.sql \
getters/g3_customer_physical_person_lookup_adt.sql \
getters/g4_customer_physical_person_lookup_adt.sql
touch getters.sql
cat getters/g1_customer_physical_person_identify_by_dni_adt.sql >> getters.sql
cat getters/g2_customer_physical_person_identify_by_cuit_adt.sql >> getters.sql
cat getters/g3_customer_physical_person_lookup_adt.sql >> getters.sql
cat getters/g4_customer_physical_person_lookup_adt.sql >> getters.sql
clean:
$(RM) banco.sql functions.sql getters.sql
<file_sep>SELECT
SUM(price)::numeric
FROM
property
WHERE
operation='Venta'
AND selling_timestamp IS NULL;<file_sep>CREATE TABLE persona (
dni integer,
nombre text
);
<file_sep>
nrodia=int(input("Ingrese un numero de dia(1 al 7): "))
if nrodia==1:
print("Lunes")
else:
if nrodia==2:
print("Martes")
else:
if nrodia==3:
print("Miercoles")
else:
if nrodia==4:
print("Jueves")
else:
if nrodia==5:
print("Viernes")
else:
if nrodia==6:
print("Sabado")
else:
if nrodia==7:
print("Domingo")
else:
if nrodia<1 or nrodia>7:
print("El numero de dia ingresado es invalido")
<file_sep>
num1=int(input("Ingrese primer numero: "))
num2=int(input("Ingrese segundo numero: "))
num3=int(input("Ingrese tercer numero: "))
if num1<num2 and num1<num3:
if num2<num3:
print("",num1,"",num2,"",num3)
else:
print("",num1,"",num3,"",num2)
elif num2<num3 and num2<num1:
if num3<num1:
print("",num2,"",num3,"",num1)
else:
print("",num2,"",num1,"",num3)
elif num3<num1 and num3<num2:
if num1<num2:
print("",num3,"",num1,"",num2)
else:
print("",num3,"",num2,"",num1)
<file_sep>
num1=int(input("Ingrese primer numero: "))
num2=int(input("Ingrese segundo numero: "))
multiplicacion=num1 * num2
print("El resultado de la multiplicacion es de: ",multiplicacion)
<file_sep># se ingresaran dos numeros para realizar una suma
declare num1=0
declare num2=0
declare suma=0
echo "Ingrese 2 numeros: "
read -p "" num1
read -p "" num2
suma=$(($num1+$num2))
echo "La suma entre esos numeros es: $suma"
exit 0
<file_sep>
print("Ingrese numeros del 1 al 100")
for i in range(1,100):
if(i%2==0):
print("",i)
<file_sep>declare base
declare height
read -p "Ingrese la base de un rectangulo: " base
read -p "Ingrese la altura de un rectangulo: " height
if (( $base > 0 && $height > 0))
then
for((i=1;i<=height;i++)) {
for((j=1;j<=base;j++)) {
echo -n "X"
}
echo ""
}
else
echo "La base y la altura deben ser mayor a 0"
fi
<file_sep>
numero=int(input("Ingrese un numero: "))
if numero != 10:
print("El numero ingresado no es 10")
<file_sep>1) Crear la Base de Datos prueba Crear la tabla person (dni integer, name text)
Crear triggers que registren las altas, bajas y modificaciones que se produzcan insertando tuplas en una tabla de log como la siguiente:
log (id serial PRIMARY KEY,timestamptz timestamptz DEFAULT now(),detail text)
Resuelto en esquema b
<file_sep>1) Desarrollar un programa que le solicite al usuario que ingrese un número entero. Determinar si el número ingresado es 10. Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
2) Desarrollar un programa que le solicite al usuario que ingrese un número entero. Determinar si el número ingresado NO es 10. Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
3) Desarrollar un programa que le solicite al usuario que ingrese su género. Las respuestas posibles son: “Masculino” y “Femenino”. Determinar si el usuario es “Femenino”. Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
4) Desarrollar un programa que solicite el ingreso de cuatro números. Determinar si el primero y el segundo son iguales, o el tercero y el cuarto son iguales. Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
5) Desarrollar un programa que determine si un usuario es de género masculino y es mayor de edad. Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
<file_sep>
declare romano
romano=""
read -p "Ingrese un numero romano: " romano
if [ $romano == "I" ]
then
echo "Valor 1"
elif [ $romano == "V" ]
then
echo "Valor 5"
elif [ $romano == "X" ]
then
echo "Valor 10"
elif [ $romano == "L" ]
then
echo "Valor 50"
elif [ $romano == "C" ]
then
echo "Valor 100"
elif [ $romano == "D" ]
then
echo "Valor 500"
elif [ $romano == "M" ]
then
echo "Valor 1000"
fi
exit 0
<file_sep>
declare genero=""
read -p "Ingrese su genero: " genero
declare edad=0
read -p "Ingrese su edad: " edad
if [[ $genero == "Masculino" && $edad > 18 ]]
then
echo "Usted es de genero masculino y es mayor de edad"
fi
exit 0
<file_sep>CREATE TABLE accounting.movement(
movement_id serial PRIMARY KEY,
movement_timestamp timestamptz DEFAULT now() NOT NULL,
movement_description text NOT NULL,
account_id integer REFERENCES accounting.account(account_id) NOT NULL,
quantity double precision CHECK(quantity != 0) NOT NULL
);
<file_sep>
num1=int(input("Ingrese primer numero: "))
num2=int(input("Ingrese segundo numero: "))
division=num1/num2
print("El resultado de la division es:",division)
<file_sep>
genero=input("Ingrese su genero: ")
edad=int(input("Ingrese su edad: "))
if genero == "Masculino" and edad > 18:
print("Su genero es masculino y es mayor de edad")
<file_sep>
declare sum=0
declare num=0
echo "ingresar numeros de forma indefinida hasta que se ingrese 0, sumarlos y mostrar su resultado"
while :
do
read -p "Ingrese numeros: " num
sum=$(( sum + num ))
(( $num > 0 ))||break
done
echo "El resultado de la suma es: $sum"
exit 0
<file_sep>CREATE TABLE person(
dni integer PRIMARY KEY,
name text NOT NULL,
surname text NOT NULL,
nationality text NOT NULL,
sex text CHECK(sex='Femenino' OR sex='Masculino')
);
CREATE TABLE contact_information(
person integer NOT NULL PRIMARY KEY REFERENCES person(dni),
mobile_phone text NOT NULL,
phone text NOT NULL,
email text NOT NULL,
address text NOT NULL
);<file_sep>
declare name=""
read -p "Ingrese su nombre: " name
declare password=0
read -p "Ingrese su contraseña: " password
if [[ $name == "Juan" && $password == <PASSWORD> ]]
then
echo "Hola Juan"
else
if [[ $name == "Pedro" && $password == <PASSWORD> ]]
then
echo "Hola <PASSWORD>"
else
echo "Usuario y/o contraseña invalidos"
fi
fi
<file_sep>length=6
i=1
j=1
for i in range(length):
for j in range(i):
print("*",end="")
print("")
print("\n")
<file_sep>
romano=str(input("Ingrese un numero romano: "))
if romano=="I":
print("Valor 1")
elif romano=="V":
print("Valor 5")
elif romano=="X":
print("Valor 10")
elif romano=="L":
print("Valor 50")
elif romano=="C":
print("Valor 100")
elif romano=="D":
print("Valor 500")
elif romano=="M":
print("Valor 1000")
else:
print("El caracter ingresado no corresponde a un numero romano")
<file_sep>
name=input("Ingrese su nombre: ")
password=int(input("Ingrese su contraseña: "))
if name=="Juan" and password==<PASSWORD>:
print("Hola <PASSWORD>")
else:
if name=="Pedro" and password==<PASSWORD>:
print("Hola <PASSWORD>")
else:
print("Usuario y/o contraseña invalidos")
<file_sep>
sum=0
print("ingresar numeros de forma indefinida hasta que se ingrese 0, sumarlos y mostrar su resultado")
while True:
num=int(input("Ingrese numeros: "))
sum=sum+num
if num == 0:
break
print("El resultado de la suma es: ",sum)
<file_sep>
genero=input("Ingrese su genero: ")
if genero == "Masculino" or genero == "Femenino":
if genero == "Femenino":
print("El genero del usuario es femenino")
else:
print("El genero ingresado no es valido")
<file_sep>SELECT
round(AVG(surface)::numeric,2)
FROM
property;<file_sep>repeat="n"
print("Ingrese 2 numeros para sumarlos y repetir esto hasta que el usuario lo disponga: ")
while True:
num1=int(input("Ingrese un numero: "))
num2=int(input("Ingrese otro numero: "))
suma=num1+num2
print("La suma de los numeros es: ",suma)
repeat=input("Desea continuar (s/n) : ")
if repeat == "n":
break
<file_sep>declare longitud
longitud=0
read -p "Ingrese la longitud del triangulo: " longitud
for((i=1;i<=longitud;i++)){
for((j=1;j<=i;j++)){
echo -n "*"
}
echo ""
}
<file_sep>declare -a array=()
declare -a array2=()
declare i
echo "Ingrese valores para el vector: "
for((i=1;i<=5;i++)){
read -p "" array[i]
}
echo ""
echo "Los valores ingresados y almacenados en otro vector multiplicando sus valores por 2 da: "
for((i=1;i<=5;i++)){
array2[i]=$((array[i]*2))
echo ${array2[i]}
}
<file_sep>declare base=0
declare height=0
echo "Ingrese base y altura de un rectangulo, deben ser mayores a 0 en caso contrario se repetira"
while :
do
read -p "Ingrese un numero: " base
read -p "Ingrese otro numero: " height
area=$(( base * height ))
(( $base <=0 && $height <=0 ))||break
done
echo "El area del rectangulo es: $area"
exit 0
<file_sep>.phony: clean
CLIENT = psql
FLAGS = -c
all: deploy
deploy: prueba11.sql
$(CLIENT) $(FLAGS) "DROP DATABASE IF EXISTS prueba11" postgres
$(CLIENT) $(FLAGS) "CREATE DATABASE prueba11" postgres
$(CLIENT) prueba11 < configuration.sql
$(CLIENT) prueba11 < 1_adt.sql
$(CLIENT) prueba11 < 2_adt.sql
prueba11.sql: \
configuration.sql \
1_adt.sql \
2_adt.sql
touch prueba11.sql
cat configuration.sql >> prueba11.sql
cat 1_adt.sql >> prueba11.sql
cat 2_adt.sql >> prueba11.sql
clean:
$(RM) prueba11.sql
<file_sep>1) Desarrolle el diagrama E/R para la base de datos inmobiliaria.
https://github.com/gbasisty/111mil/blob/master/baseDatos/inmobiliaria_adt.sql
2) Desarrolle el diagrama E/R para el Ejercicio 1 del Apunte 2 de Base de Datos:
Crear una base de datos relacional para un instituto de formación profesional. Se debe registrar alumnos, docentes y cursos. Determinar qué alumnos está inscritos en cada curso, y qué docentes están dictando cada curso.
Respecto a los alumnos se desea saber:
-Nombre (obligatorio)
-Apellido (obligatorio)
-DNI (obligatorio y único)
-Fecha de Nacimiento (obligatorio)
-Dirección (obligatorio)
-Teléfono
-eMail
-Fecha de Inscripción (obligatorio)
-Número de legajo (obligatorio y único)
-Qué curso está realizando
Respecto a los docentes se desea saber:
-Nombre (obligatorio)
-Apellido (obligatorio)
-DNI (obligatorio y único)
-Fecha de Nacimiento (obligatorio)
-Dirección (obligatorio)
-Teléfono
-eMail
-Fecha de contratación (obligatorio)
-CUIL (obligatorio y único)
-Honorarios (obligatorio, y debe ser mayor que cero)
-De qué curso es docente
Respecto a los cursos se desea saber:
-Nombre del curso (obligatorio y único)
-Descripción (obligatorio)
-Código del curso (obligatorio y único)
-Costo del curso (obligatorio, y debe ser mayor que cero)
3) Crear el modelo de datos para una agencia de alquiler de vehículos.
Se desea registrar respecto a los clientes:
-Nombre (obligatorio)
-Apellido (obligatorio)
-Fecha de nacimiento (obligatorio)
-DNI (obligatorio y único)
-Género (obligatorio, Masculino o Femenino)
-Dirección
-Teléfono
-Correo electrónico
Se desea registrar respecto a los vehículos:
-Patente (obligatorio y único)
-Marca (obligatorio)
-Modelo (obligatorio)
-Tipo (obligatorio, Auto o Utilitario)
-Fecha de adquisición (obligatorio)
Respecto a los alquileres se desea saber:
-Que cliente alquiló que vehículo
-En qué momento lo retiró (obligatorio)
-En qué momento lo devolvió (nulo si aún no lo devuelve)
-Cuál es el costo total del alquiler (debe ser mayor a cero)
<file_sep>CREATE SCHEMA a;
CREATE SCHEMA b;
<file_sep>
nrodia=int(input("Ingrese un numero de dia(1 al 7): "))
if nrodia==1:
print("Lunes")
elif nrodia==2:
print("Martes")
elif nrodia==3:
print("Miercoles")
elif nrodia==4:
print("Jueves")
elif nrodia==5:
print("Viernes")
elif nrodia==6:
print("Sabado")
elif nrodia==7:
print("Domingo")
elif nrodia<1 or nrodia>7:
print("El numero de dia ingresado no es valido")
<file_sep>
num1=int(input("Ingrese primer numero: "))
num2=int(input("Ingrese segundo numero: "))
num3=int(input("Ingrese tercer numero: "))
num4=int(input("Ingrese cuarto numero: "))
num5=int(input("Ingrese quinto numero: "))
print("\nNumeros ordenados de forma descendente",num5,num4,num3,num2,num1)
<file_sep>
num1=int(input("Ingrese el primer numero: "))
num2=int(input("Ingrese el segundo numero: "))
num3=int(input("Ingrese el tercer numero: "))
num4=int(input("Ingrese el cuarto numero: "))
if num1 == num2 and num3 == num4:
print("El primer y el segundo numero son iguales o el tercero y el cuarto son iguales")
<file_sep>
echo "Ingrese 2 numeros: "
num1=0.0
read -p "Ingrese primer numero: " num1
num2=0.0
read -p "Ingrese segundo numero: " num2
division=$[$num1 / $num2]
echo "El resultado de la division es: $division"
exit 0
<file_sep>declare i
i=1
echo "Numeros pares del 1 al 100: "
for((i=1;i<=100;i++)){
if((i%2==0))
then
echo "$i"
fi
}
<file_sep>
print("Ingrese base y altura de un rectangulo, deben ser mayores a 0 en caso contrario se repetira")
while True:
base=int(input("Ingrese la base: "))
height=int(input("Ingrese la altura: "))
area=base*height
if base>0 and height>0:
break
print("El area del rectangulo es: ",area)
<file_sep>
INSERT INTO b.products VALUES('polenta','maiz con especias','23');
DELETE FROM b.products WHERE price = '23';
UPDATE b.products SET name = 'tomate' WHERE price = '23';
SELECT * FROM b.products;<file_sep>
length=6
nlength=length-1
i=1
j=1
for i in range(length):
for j in range(i):
print("X",end="")
print("")
for i in range (1,length):
for j in range(i,nlength):
print("X",end="")
print("")
<file_sep>
declare genero=""
read -p "Ingrese su genero: " genero
if [[ "$genero" == "Masculino" || $genero == "Femenino" ]]
then
if [[ $genero = "Femenino" ]]
then
echo "El genero ingresado es femenino"
fi
else
echo "El genero ingresado no es valido"
fi
exit 0
<file_sep>
declare nrodia
nrodia=0
read -p"Ingrese un numero de dia de la semana: " nrodia
case $nrodia in
1)
echo "Lunes"
;;
2)
echo "Martes"
;;
3)
echo "Miercoles"
;;
4)
echo "Jueves"
;;
5)
echo "Viernes"
;;
6)
echo "Sabado"
;;
7)
echo "Domingo"
;;
*)
echo "No es un numero de dia valido"
;;
esac
exit 0
<file_sep>1) Desarrollar un programa que le solicite al usuario que ingrese un número entero. Determinar si el número ingresado es múltiplo de 2 o no (utilizar el operador de módulo). Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
2) Desarrollar un programa que le solicite al operador que ingrese su nombre y contraseña. Las personas autorizadas a utilizarlo son Juan (identificado con la contraseña <PASSWORD>) y Pedro (identificado con la contraseña <PASSWORD>). Si el operador es un usuario autorizado, saludarlo por su nombre, sino, salir del programa mostrando un mensaje de error. Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
<file_sep>Ejercicio1:Desarrollar un software que le solicite al usuario que ingrese el nombre de su barrio, la dirección postal de la comisaría más cercana, y las entrecalles. Luego mostrar un mensaje por pantalla similar a “La comisaría de Banfield se encuentra en la calle Maipú 133 entre Alsina y Belgrano”.
Ejercicio2: Desarrollar un software que le solicite al usuario sus datos personales, a saber: DNI, nombre y apellido, dirección y teléfono. Mostrar los datos por pantalla. Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
Ejercicio3: Desarrollar un software que le solicite al usuario el ingreso de 5 números, y mostrarlos en el orden inverso al ingresado. Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
Ejercicio4: Desarrollar un software que le solicite al usuario el ingreso de 2 números y le muestre al usuario el resultado de la suma de esos números. Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
Ejercicio5: Desarrollar un software que le solicite al usuario el ingreso de 2 números y le muestre al usuario el resultado de la resta de esos números. Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
Ejercicio6: Desarrollar un software que le solicite al usuario el ingreso de 2 números y le muestre al usuario el resultado de la resta de esos números. Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
Ejercicio7:Desarrollar un software que le solicite al usuario el ingreso de 2 números y le muestre al usuario el resultado de la división de esos números. Presentar diagrama de flujo, pseudocódigo y código fuente funcionando en BASH y Python.
<file_sep>SELECT
concat(name,' ',surname) "Nombre",
cuit "CUIT",
address "Dirección"
FROM
customer.customer
UNION
SELECT
name,
cuit,
address
FROM
provider.provider;
<file_sep>name=input("Ingrese su nombre: ")
surname=input("Ingrese su apellido: ")
dni=int(input("Ingrese su numero de dni: "))
direction=input("Ingrese su direccion: ")
telephone=int(input("Ingrese su numero de telefono: "))
print("El señor/a con nombre",name,"apellido",surname,"dni",dni,"direccion",direction,"y telefono",telephone,"se ha registrado")
<file_sep>
declare carac
carac=""
read -p"Ingrese un caracter cualquiera(consonante,vocal,numero): " carac
case $carac in
"a")
echo "Es una vocal"
;;
"e")
echo "Es una vocal"
;;
"i")
echo "Es una vocal"
;;
"o")
echo "Es una vocal"
;;
"u")
echo "Es una vocal"
;;
"b")
echo "Es una consonante"
;;
"c")
echo "Es una consonante"
;;
"d")
echo "Es una consonante"
;;
"f")
echo "Es una consonante"
;;
"g")
echo "Es una consonante"
;;
"h")
echo "Es una consonante"
;;
"0")
echo "Es un numero"
;;
"1")
echo "Es un numero"
;;
"2")
echo "Es un numero"
;;
"3")
echo "Es un numero"
;;
"4")
echo "Es un numero"
;;
"5")
echo "Es un numero"
;;
"6")
echo "Es un numero"
;;
"7")
echo "Es un numero"
;;
"8")
echo "Es un numero"
;;
"9")
echo "Es un numero"
;;
esac
exit 0
<file_sep>CREATE TABLE accounting.account(
account_id serial PRIMARY KEY,
owner_cuit text REFERENCES customer.person(cuit) NOT NULL,
opening_date timestamptz NOT NULL DEFAULT now(),
account_type account_type NOT NULL DEFAULT 'Caja de Ahorro',
authorized_uncovered_balance double precision DEFAULT 0 CHECK(authorized_uncovered_balance <= 0) NOT NULL,
balance double precision DEFAULT 0 CHECK(balance >= authorized_uncovered_balance) NOT NULL
);
<file_sep>.phony: clean
CLIENT = psql
FLAGS = -c
all: deploy
deploy: comercio.sql
$(CLIENT) $(FLAGS) "DROP DATABASE IF EXISTS comercio" postgres
$(CLIENT) $(FLAGS) "CREATE DATABASE comercio" postgres
$(CLIENT) comercio < comercio.sql
comercio.sql: \
configuration.sql \
customer/customer_adt.sql \
provider/provider_adt.sql
touch comercio.sql
cat configuration.sql >> comercio.sql
cat customer/customer_adt.sql >> comercio.sql
cat provider/provider_adt.sql >> comercio.sql
clean:
$(RM) comercio.sql
<file_sep>
echo "Ingrese 2 numeros: "
declare num1=0
read -p "" num1
declare num2=0
read -p "" num2
declare multiplicacion=0
multiplicacion=$(($num1 * $num2))
echo "El resultado de la multiplicacion es de: $multiplicacion"
exit 0
<file_sep>1) Crear la Base de Datos banco del modelo XML de banco.xml
<file_sep>#!/bin/bash
declare -i dni=0
read -p"Ingrese su numero de dni: " dni
declare name=""
read -p"Ingrese su nombre: " name
declare surname=""
read -p"Ingrese su apellido: " surname
declare direction=""
read -p"Ingrese su direccion: " direction
declare -i telephone=0
read -p"Ingrese su numero de telefono: " telephone
echo "El señor/a $name $surname con dni $dni telefono $telephone y direccion $direction se ha registrado"
exit 0
<file_sep>SELECT
c.region_name,
count(operation)
FROM
property a
NATURAL INNER JOIN seller b
NATURAL INNER JOIN region c
WHERE
b.seller_name='Luisa' AND
b.seller_surname='Estevez'
GROUP BY 1
ORDER BY 2 DESC
LIMIT 1;<file_sep>SELECT
property_id,
price
FROM
property a
NATURAL INNER JOIN seller b
WHERE
seller_name='Pedro' AND
seller_surname='Iriarte'
ORDER BY price DESC;<file_sep>
number=int(input("Ingrese un numero: "))
i=1
j=1
for i in range(number,number+1):
for j in range(1,11):
table=i*j
print("",i,"*",j,"=",table)
<file_sep>
declare nrodia
nrodia=0
read -p "Ingrese un numero de dia(1 al 7): " nrodia
if (( $nrodia == 1 ))
then
echo "Lunes"
elif (( $nrodia == 2 ))
then
echo "Martes"
elif (( $nrodia == 3 ))
then
echo "Miercoles"
elif (( $nrodia == 4 ))
then
echo "Jueves"
elif (( $nrodia == 5 ))
then
echo "Viernes"
elif (( $nrodia == 6 ))
then
echo "Sabado"
elif (( $nrodia == 7 ))
then
echo "Domingo"
else
if (( $nrodia<1 || $nrodia>7 ))
then
echo "El numero de dia ingresado es invalido"
fi
fi
exit 0
<file_sep>SELECT
b.region_name,
a.property_type_name,
max(price)
FROM
property c
NATURAL INNER JOIN property_type a
NATURAL INNER JOIN region b
WHERE
region_name='Lleida'
GROUP BY 1,2
ORDER BY 3 DESC
LIMIT 1;<file_sep>CREATE SCHEMA customer;
CREATE SCHEMA provider;
<file_sep>SELECT
b.seller_name,
b.seller_surname,
b.seller_dni,
max(price)
FROM
seller b
NATURAL INNER JOIN property a
GROUP BY 1,2,3
ORDER BY 4 ASC
LIMIT 1;<file_sep>i=1
sum=0
for i in range(1,101):
sum=sum+i
print("La suma de numeros del 1 al 100 da: ",sum)
<file_sep>declare -i numero=1
echo "Ingrese numeros hasta que alguno sea mayor a 100"
while :
do
read -p "Ingrese numero: " numero
(( $numero <= 100 ))||break
done
exit 0
<file_sep>DROP VIEW vw_selling_report;
CREATE VIEW vw_selling_report AS
SELECT
a.property_id "ID Propiedad",
c.property_type_name "Tipo de Propiedad",
a.price "Precio",
a.creation_timestamp "Fecha de Ingreso",
a.selling_timestamp "Fecha de Venta",
concat(b.seller_surname,' ',b.seller_name) "Vendedor",
date_part('day', a.selling_timestamp - a.creation_timestamp) "Periodo de Venta en Dias"
FROM
property a
NATURAL INNER JOIN seller b
NATURAL INNER JOIN property_type c
WHERE
operation='Venta'
ORDER BY 7 DESC;
SELECT
"Tipo de Propiedad",
round(avg("Periodo de Venta en Dias"))
FROM
vw_selling_report
GROUP BY 1
ORDER BY 2 ASC
LIMIT 1;
<file_sep>declare length
length=5
echo -e "\n"
for((i=1;i<=length;i++)) {
for((j=1;j<=i;j++))
{
echo -n "*"
}
echo ""
}
for((i=1;i<=length;i++)) {
for((j=i;j<=length-1;j++)) {
echo -n "*"
}
echo ""
}
exit 0
<file_sep>CREATE TABLE customer.physical_person(
dni text UNIQUE NOT NULL,
surname text NOT NULL,
name text NOT NULL,
birthday timestamptz NOT NULL,
cuit text PRIMARY KEY REFERENCES customer.person(cuit)
);
<file_sep>CREATE SCHEMA b;<file_sep>
declare romano
romano=""
read -p "Ingrese una letra que corresponda a un numero romano(I,V,X,L,C,D,M): " romano
case $romano in
"I")
echo "Valor 1"
;;
"V")
echo "Valor 5"
;;
"X")
echo "Valor 10"
;;
"L")
echo "Valor 50"
;;
"C")
echo "Valor 100"
;;
"D")
echo "Valor 500"
;;
"M")
echo "Valor 1000"
;;
*)
echo "No corresponde a un numero romano valido"
;;
esac
exit 0
<file_sep>INSERT INTO b.person VALUES('17','yo','tu','28-03-1989');
DELETE FROM b.person WHERE dni = '17';
UPDATE b.person SET name = 'al' WHERE dni = '17';
| e8c4603cf7ea0f8888860838f0f2d167bae782fb | [
"SQL",
"Markdown",
"Makefile",
"Python",
"Shell"
] | 126 | Shell | Mtorrents/111mils | 6b7b91909d4a26c2ae64f023da231ee0bd0226e7 | b488bfe4416a4736592981ed91817844f9ccf325 |
refs/heads/master | <repo_name>JavaEdge/AlgorithmStudy<file_sep>/src/graph/depthFirstSearch.js
/**
* 깊이 우선 탐색
*
* 풀이 과정:
*
출발 노드를 OPEN 에 삽입;
while not empty(OPEN) do
n = OPEN 의 제일 앞 노드;
n을 OPEN 에서 제거하여 CLOSED 에 넣음;
if depth(n) < 깊이 제한 then
노드 n을 확장 하여 모든 후계노드를 생성;
생성 후계 노드들에 부모노드 n을 가리키는 포인터를 첨부;
if 후계노드 중 목표노드가 존재 then
그 후계노드로부터 포인터를 역으로 추적하여 풀이경로 구성;
return 탐색성공;
else
후계노드를 OPEN의 앞에 넣음;
end-if;
end-if;
end-while;
return 탐색 실패;
*
*/
// 인접리스
var get_adjacency_arr = function(vertex_arr, edge_arr) {
var adjacency_arr = [];
var v_len = vertex_arr.length;
var e_len = edge_arr.length;
for(var i = 0; i < v_len; i++){
var adjacency = [];
for(var j = 0; j < e_len; j++){
if (vertex_arr[i] === edge_arr[j][0]) {
adjacency.push(edge_arr[j][1]);
} else if (vertex_arr[i] === edge_arr[j][1]) {
adjacency.push(edge_arr[j][0]);
}
}
adjacency_arr.push(adjacency);
}
return adjacency_arr;
};
// 경로 구하기
var get_path = function(current, child_node, CLOSED){
// 경로 추적.
var parent = current;
var path = [];
path.push(child_node.v);
while(parent){
path.push(parent.v);
var closed_len = CLOSED.length;
if (parent.p !== null) {
for(var j = 0; j < closed_len; j++) {
var closed_data = CLOSED[j];
if (closed_data.v === parent.p) {
parent = closed_data;
break;
}
}
}else {
parent = null;
}
}
return path.reverse();
};
// 깊이 우선 탐색
var f_dfs = function(data) {
var adjacency_arr = get_adjacency_arr(data.vertex_arr, data.edge_arr);
var OPEN = [{v: data.vertex_arr[0], p: null}];
var CLOSED = [];
var path = [];
while(OPEN) {
var current = OPEN.pop();
CLOSED.push(current);
if(!current) {
OPEN = null;
continue;
}
console.log("search node: " + current.v);
var children_arr = adjacency_arr[current.v];
var children_len = children_arr.length;
for (var i = 0; i < children_len; i++) {
var child_node = { v: children_arr[i], p: current.v};
if(data.result === data.text_arr[child_node.v]) {
path = get_path(current, child_node, CLOSED);
OPEN = null;
break;
} else {
var closed_len = CLOSED.length;
var is_closed = false;
for(var j = 0; j < closed_len; j++) {
var closed_data = CLOSED[j];
if (closed_data.v === child_node.v) {
is_closed = true;
break;
}
}
if (!is_closed) {
OPEN.push(child_node);
}
}
}
}
return path;
};
// 테스트 데이터
var test_data = {};
test_data.result = "phone"; // 목표값
test_data.text_arr = ["sky", "apple", "game", "computer", "phone", "google"]; // 실제 노드의 값
test_data.vertex_arr = [ 0, 1, 2, 3, 4, 5]; // 간단하게 추상화한 노드리스트
test_data.edge_arr = [[0, 1], [1, 2], [2, 3], [1, 3], [2, 4], [3, 5]]; // 엣지리스트
/**
* 0 - 1 - 2 - 4
* ㄴ ㄴ 3 - 5
*
*/
var path = f_dfs(test_data);
console.log(path);<file_sep>/README.md
# 简单算法学习 :octocat:
## 冒泡排序
原理:
1. 比较相邻的元素。如果第一个比第二个大,就交换他们两个。
1. 对每一对相邻元素作同样的工作,从开始第一对到结尾的最后一对。这步做完后,最后的元素会是最大的数。
1. 针对所有的元素重复以上的步骤,除了最后一个。
1. 持续每次对越来越少的元素重复上面的步骤,直到没有任何一对数字需要比较。
\ | 最坏 | 平均 | 最优
----- | ----- | ----- | -----
时间复杂度 | O(n2) | O(n2) | O(n2) |
空间复杂度 | O(1)
## 插入排序
原理:
1. 从第一个元素开始,该元素可以认为已经被排序
1. 取出下一个元素,在已经排序的元素序列中从后向前扫描
1. 如果该元素(已排序)大于新元素,将该元素移到下一位置
1. 重复步骤3,直到找到已排序的元素小于或者等于新元素的位置
1. 将新元素插入到该位置后
1. 重复步骤2~5
\ | 最坏 | 平均 | 最优
----- | ----- | ----- | -----
时间复杂度 | O(n2) | O(n2) | O(n) |
空间复杂度 | O(1)
## 选择排序
原理:
它的工作原理是每一次从待排序的数据元素中选出最小(或最大)的一个元素,存放在序列的起始位置,直到全部待排序的数据元素排完。 选择排序是不稳定的排序方法。
\ | 最坏 | 平均 | 最优
----- | ----- | ----- | -----
时间复杂度 | O(n2) | O(n2) | O(n2) |
空间复杂度 | O(1)
## 快速排序
原理:
1. 从数列中挑出一个元素,称为"基准"(pivot),
1. 重新排序数列,所有比基准值小的元素摆放在基准前面,所有比基准值大的元素摆在基准后面(相同的数可以到任何一边)。在这个分区结束之后,该基准就处于数列的中间位置。这个称为分区(partition)操作。
1. 递归地(recursively)把小于基准值元素的子数列和大于基准值元素的子数列排序。
\ | 最坏 | 平均 | 最优
----- | ----- | ----- | -----
时间复杂度 | O(n2) | O(nlogn) | O(nlogn) |
空间复杂度 | O(1)
## 归并排序
原理如:
递归法(Bottom-up)
1. 将序列每相邻两个数字进行归并操作,形成 {\displaystyle ceil(n/2)} {\displaystyle ceil(n/2)}个序列,排序后每个序列包含两/一个元素
1. 若此时序列数不是1个则将上述序列再次归并,形成 {\displaystyle ceil(n/4)} {\displaystyle ceil(n/4)}个序列,每个序列包含四/三个元素
1. 重复步骤2,直到所有元素排序完毕,即序列数为1
\ | 最坏 | 平均 | 最优
----- | ----- | ----- | -----
时间复杂度 | O(nlogn) | O(nlogn) | O(n) |
空间复杂度 | O(n)
## 堆排序
原理:
1. 最大堆调整(Max_Heapify):将堆的末端子节点作调整,使得子节点永远小于父节点
1. 创建最大堆(Build_Max_Heap):将堆所有数据重新排序
1. 堆排序(HeapSort):移除位在第一个数据的根节点,并做最大堆调整的递归运算
\ | 最坏 | 平均 | 最优
----- | ----- | ----- | -----
时间复杂度 | O(nlogn) | O(nlogn) | O(nlogn)
空间复杂度 | O(1)
## 数组容器接水问题
1. 双指针问题。
1. 从左右相互出发,如果一方的最大值小于等于另一方的最大值,就从小的一方为基准,把指针移动中的差异累计,直到中间遇到比大的一方大或相同的数为止。
1. 遇到的更大或相同的数为更新的最大值,再与另一方比较。
1. 重复 2 ~ 3过程, 直到左右两个指针不换位。
\ | 最坏 | 平均 | 最优
----- | ----- | ----- | -----
时间复杂度 | O(n) | O(n) | O(n)
空间复杂度 | O(1)
## 2分查找 ( 折半查找 )
前提条件必须采用顺序储蓄结构, 必须按关键字大小有序排列
1. 折半查找
1. 时间复杂度为logn
## 二叉树后续遍历 LRD (Postorder Traversal)
1. 先遍历左子树
1. 然后遍历右子树
1. 最后遍历根节点
## 二叉树中续遍历 LDR (Inorder Traversal)
1. 先遍历左子树
1. 然后遍历根节点
1. 最后遍历右子树
## 二叉树中续遍历 DLR (Preorder Traversal)
1. 先遍历根节点
1. 然后遍历左子树
1. 最后遍历右子树
## 二元查找树:
它首先要是一棵二元树,在这基础上它或者是一棵空树;或者是具有下列性质的二元树
1. 若左子树不空,则左子树上所有结点的值均小于它的父结点的值;
2. 若右子树不空,则右子树上所有结点的值均大于等于它的根结点的值;
3. 左、右子树也分别为二元查找树
## 红黑树
1. 每个节点为红色或黑色
1. 根节点为黑色
1. 每个空(nil)节点为黑色
1. 每个红色节点的两个子节点为黑色
1. 每个节点到每个叶节点的所有路径都包含相同数量的黑色节点
<file_sep>/src/graph/dijkstraPathFinding.js
/**
* Dijkstra最短路径
*
*
*/
let keys = [];
let open_arr = [];
let closed = [];
let sortes = {};
let total = 0;
let end_key = null;
let now = null;
let graph = null;
let setStartNode = (key) => {
// console.log("start key => " + key);
let idx = keys.indexOf(key);
if( idx >= 0) {
for(let i = 0; i < keys.length; i++) {
if ( idx === i) {
open_arr.push(key);
sortes[key] = { p: null, path: 0};
} else {
sortes[keys[i]] = { p: null, path: -1};
}
}
// console.log("sortes => " + JSON.stringify(sortes));
// console.log("open_arr => " + JSON.stringify(open_arr));
// console.log("closed => " + JSON.stringify(closed));
} else {
console.log("해당 키가 없다.");
return;
}
};
let setEndNode = (key) => {
end_key = key;
};
let reverse_path = () => {
let sortest_path = [];
let key = end_key;
while(key) {
sortest_path.push(key);
key = sortes[key].p
}
sortest_path = sortest_path.reverse();
console.log(JSON.stringify(sortest_path));
};
let getPath = (start, end ) => {
open_arr = [];
closed = [];
total = 0;
sortes = {};
end_key = null;
now = null;
setStartNode(start);
setEndNode(end);
// console.log("map : " + JSON.stringify(graph));
// console.log("open_arr : " + JSON.stringify(open_arr));
while(open_arr.length > 0) {
now = open_arr.shift();
if(closed.indexOf(now) >= 0) continue;
let childKeys = Object.keys(graph[now]);
// console.log("keys => " + JSON.stringify(childKeys));
for(let i = 0; i < childKeys.length; i++){
let path = sortes[now].path + graph[now][childKeys[i]];
// console.log("chid : " + childKeys[i] + ", path" + path);
if(sortes[childKeys[i]].path === -1 || sortes[childKeys[i]].path > path){
sortes[childKeys[i]].p = now;
sortes[childKeys[i]].path = path
}
open_arr.push(childKeys[i]);
}
closed.push(now);
}
// console.log(JSON.stringify(sortes));
if(sortes[end_key].path >= 0) {
reverse_path();
console.log("最短距离为" + sortes[end_key].path);
}else{
console.log("没有最短距离");
}
}
let dijkstraSortestPath = (map) => {
keys = Object.keys(map);
// console.log(JSON.stringify(keys));
graph = map;
};
var map = {a:{b:3,c:1},b:{a:2,c:1},c:{a:4,b:1}};
dijkstraSortestPath(map);
getPath('a', 'b'); // => ['a', 'c', 'b']
getPath('a', 'c'); // => ['a', 'c']
getPath('b', 'a'); // => ['b', 'a']
getPath('b', 'c', 'b'); // => ['b', 'c', 'b']
getPath('c', 'a', 'b'); // => ['c', 'b', 'a', 'c', 'b']
getPath('c', 'b', 'a'); // => ['c', 'b', 'a']<file_sep>/src/graph/breadthFirstSearch.js
/**
* Breadth First Search
*
* 解题过程:
* 1. 把0节点添加到queue
2. 读取queue的第一个节点
3. 并把当前节点的左右节点添加到queue
4. 重复2~3, 直到queue没有任何节点
*
*/
var queue = [];
var breadth_first_search = function( search_data ){
var node = queue.shift();
console.log(node.value);
if(node.value === search_data ){
console.log("已找到。结束程序。");
return;
}
if(node.left) {
queue.push(node.left);
}
if(node.right) {
queue.push(node.right);
}
if(queue.length === 0){
console.log("没有结果, 结束程序");
return;
}
breadth_first_search( search_data );
};
var a = {
value: 10
};
var b = {
value: 8
};
var c = {
value: 7
};
var d = {
value: 5
};
var e = {
value: 11
};
var f = {
value: 12
}
a.left = b;
a.right = c;
b.left = d;
b.left = e;
c.right = f;
queue.push(a);
var path = breadth_first_search(31);<file_sep>/src/graph/uniformCostSearch.js
/**
* 균일비용 탐색
*
* 풀이 과정:
*
출발 노드를 OPEN 에 삽입, 출발노드의 경로비용은 0;
while not empty(OPEN) do
n = OPEN 의 제일 앞 노드;
n을 OPEN 에서 제거하여 CLOSED 에 넣음;
if depth(n) < 깊이 제한 then
노드 n을 확장 하여 모든 후계노드를 생성;
생성 후계 노드들에 부모노드 n을 가리키는 포인터를 첨부;
if 후계노드 중 목표노드가 존재 then
그 후계노드로부터 포인터를 역으로 추적하여 풀이경로 구성;
return 탐색성공;
else
후계노드를 OPEN의 앞에 넣음;
end-if;
end-if;
end-while;
return 탐색 실패;
*
*/ | 73ebd3bc92ffe70903937cb2b394880add46c07f | [
"JavaScript",
"Markdown"
] | 5 | JavaScript | JavaEdge/AlgorithmStudy | cf3d4f6a3c19cc9bab9cc04b86c71561f67c42e8 | bf0c40926b44d07ba2117f9cf6b9929fb6afff85 |
refs/heads/master | <repo_name>rs-aleksey/rsui-tree<file_sep>/src/tree/layout/TreeLayoutNodeExit.js
import React, { Component } from 'react';
import TreeLayoutNodeContainer from './TreeLayoutNodeContainer';
import TreeLayoutNodeCard from './TreeLayoutNodeCard';
import TreeLayoutVerticalLine from './TreeLayoutVerticalLine';
import styled from 'styled-components';
const TreeLayoutNodeContainerStyled = styled(TreeLayoutNodeContainer)`
flex: auto;
`;
export default class TreeLayoutNodeExit extends Component {
render() {
return (
<TreeLayoutNodeContainerStyled>
<TreeLayoutVerticalLine />
<TreeLayoutNodeCard backgroundColor="#CCC">exit</TreeLayoutNodeCard>
</TreeLayoutNodeContainerStyled>
);
}
}
<file_sep>/src/tree/layout/TreeLayoutNodeCard.js
import styled from 'styled-components';
const TreeLayoutNodeCard = styled.div`
border: 2px solid #000;
padding: 20px;
background-color: #FFF;
align-self: center;
${props => props.backgroundColor ? `
background-color: ${props.backgroundColor};
` : ''}
`;
TreeLayoutNodeCard.displayName = 'TreeLayoutNodeCard';
export default TreeLayoutNodeCard;
<file_sep>/src/tree/TreeNodeSegment.js
import React, { Component } from 'react';
import TreeNodes from './TreeNodes';
import TreeLayoutNodeCard from './layout/TreeLayoutNodeCard';
import TreeLayoutNodeContainer from './layout/TreeLayoutNodeContainer';
import TreeLayoutVerticalLine from './layout/TreeLayoutVerticalLine';
import TreeLayoutNodeSegmentSplitHeader from './layout/TreeLayoutNodeSegmentSplitHeader';
import TreeLayoutNodeSegmentSplitFooter from './layout/TreeLayoutNodeSegmentSplitFooter';
import {
NODE_TYPE_SEGMENT_CLOSED,
NODE_TYPE_SEGMENT_OPENED,
} from './constants';
import TreeLayoutNodeExit from './layout/TreeLayoutNodeExit';
export default class TreeNodeSegment extends Component {
get shouldRenderChildren() {
const { node: { type } } = this.props;
if (type === NODE_TYPE_SEGMENT_OPENED) {
return true;
}
return this.hasChildren;
}
get hasChildren() {
const { node: { children } } = this.props;
if (children && (children[0] || children[1])) {
return true;
}
return false;
}
get shouldRenderFooter() {
return this.props.node.type === NODE_TYPE_SEGMENT_CLOSED;
}
get shouldRenderExit() {
const {
node: { type },
exitRendered,
delegatedExitRender,
} = this.props;
if (exitRendered || delegatedExitRender) {
return false;
}
if (type === NODE_TYPE_SEGMENT_OPENED) {
return false;
}
if (this.hasThirdChild) {
return false;
}
return true;
}
get hasThirdChild() {
const {
node: {
type,
children,
},
} = this.props;
if (type === NODE_TYPE_SEGMENT_OPENED) {
return false;
}
if (!children || !children[2]) {
return false;
}
return true;
}
get hasThirdChild() {
return !!this.thirdChild;
}
get thirdChild() {
const {
node: { children },
} = this.props;
return children && children[2];
}
render() {
const {
node,
node: { children },
exitRendered,
delegatedExitRender,
} = this.props;
return (
<TreeLayoutNodeContainer>
<TreeLayoutNodeCard backgroundColor="#AFA">segment</TreeLayoutNodeCard>
<TreeLayoutVerticalLine />
<TreeLayoutNodeSegmentSplitHeader />
{this.shouldRenderChildren && (
<TreeNodes
parentNode={node}
childrenNodes={children}
parentSegment={node}
exitRendered={exitRendered || this.shouldRenderExit}
delegatedExitRender={delegatedExitRender || this.hasThirdChild}
/>
)}
{this.shouldRenderFooter && (
<TreeLayoutNodeSegmentSplitFooter />
)}
{this.hasThirdChild && (
<TreeNodes
parentNode={node}
childrenNodes={[this.thirdChild]}
parentSegment={node}
exitRendered={exitRendered || this.shouldRenderExit}
delegatedExitRender={delegatedExitRender}
/>
)}
{this.shouldRenderExit && (
<TreeLayoutNodeExit />
)}
</TreeLayoutNodeContainer>
);
}
}
<file_sep>/src/tree/layout/TreeLayoutNodeSegmentSplitFooter.js
import React, { Component } from 'react';
import TreeLayoutVerticalLine from './TreeLayoutVerticalLine';
import TreeLayoutHalfHorizontalLine from './TreeLayoutHalfHorizontalLine';
import TreeLayoutNodeSingleColumn from './TreeLayoutNodeSingleColumn';
import styled from 'styled-components';
const TreeLayoutNodeSingleColumnStyled = styled(TreeLayoutNodeSingleColumn)`
flex: 1;
`;
export default class TreeLayoutNodeSegmentSplitFooter extends Component {
render() {
return (
<TreeLayoutNodeSingleColumnStyled>
<TreeLayoutHalfHorizontalLine />
<TreeLayoutVerticalLine />
</TreeLayoutNodeSingleColumnStyled>
);
}
}
<file_sep>/src/tree/layout/TreeLayoutNodeSingleColumn.js
import styled from 'styled-components';
const TreeLayoutNodeSingleColumn = styled.div`
display: inline-flex;
justify-content: center;
flex-direction: column;
`;
TreeLayoutNodeSingleColumn.displayName = 'TreeLayoutNodeSingleColumn';
export default TreeLayoutNodeSingleColumn;
<file_sep>/README.md
RSUI Path Builder Tree Demo

<file_sep>/src/tree/layout/TreeLayoutHalfHorizontalLine.js
import styled from 'styled-components';
const TreeLayoutHalfHorizontalLine = styled.div`
width: calc(50% + 2px);
height: 2px;
background-color: #000;
margin-left: calc(25% - 1px);
`;
TreeLayoutHalfHorizontalLine.displayName = 'TreeLayoutHalfHorizontalLine';
export default TreeLayoutHalfHorizontalLine;
<file_sep>/src/tree/constants.js
export const NODE_TYPE_EMAIL = 'NODE_TYPE_EMAIL';
export const NODE_TYPE_SEGMENT_OPENED = 'NODE_TYPE_SEGMENT_OPENED';
export const NODE_TYPE_SEGMENT_CLOSED = 'NODE_TYPE_SEGMENT_CLOSED';
export const NODE_TYPE_TIMING = 'NODE_TYPE_TIMING';
<file_sep>/src/tree/layout/TreeLayoutNodeTwoColumnsContainer.js
import styled from 'styled-components';
const TreeLayoutNodeTwoColumnsContainer = styled.div`
display: inline-flex;
`;
TreeLayoutNodeTwoColumnsContainer.displayName = 'TreeLayoutNodeTwoColumnsContainer';
export default TreeLayoutNodeTwoColumnsContainer;
| 544855f0a528d6dbd4400a8f7619d8179fbf3fae | [
"JavaScript",
"Markdown"
] | 9 | JavaScript | rs-aleksey/rsui-tree | c4d7fc483654dbedc7240e81b6a7b8d8bf5ff31d | e59baf93d1cdf54c55b595b24e561ce684eebe10 |
refs/heads/master | <file_sep>path.variable.kotlin_bundled=C\:\\Program Files\\JetBrains\\IntelliJ IDEA 2018.2.6\\plugins\\Kotlin\\kotlinc
path.variable.maven_repository=C\:\\Users\\40682\\.m2\\repository
javac2.instrumentation.includeJavaRuntime=false<file_sep>import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
import java.sql.DriverManager;
import java.sql.Statement;
import java.text.SimpleDateFormat;
@WebServlet(name = "AddAccount")
public class AddAccount extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
HttpSession session=request.getSession();
String student_id=request.getParameter("student_id");
String password=request.getParameter("password");
String nickname=request.getParameter("nickname");
String email=request.getParameter("email");
String wechat=request.getParameter("wechat");
String whatsapp=request.getParameter("whatsapp");
String qq=request.getParameter("qq");
if(student_id.equals("")||password.equals("")){ response.setHeader("refresh", "3;url=register.jsp"); }
try {
Class.forName("com.mysql.jdbc.Driver");
Statement stm = DriverManager.getConnection("jdbc:mysql://localhost:3306/peproject", "root", "admin").createStatement();
java.util.Date d=new java.util.Date();
SimpleDateFormat sdf=new SimpleDateFormat("yyyy-MM-dd hh:mm:ss");
String da= sdf.format(d);//register_time
String sql =
"Insert into peproject.user(student_id,password,nickname,email,wechat,whatsapp,qq,register_date) \n" +
"values('"+ student_id+"','"+password+"','"+nickname+"','"+email+"','"+wechat+"','"+whatsapp+"','"+qq+"','"+da+"');";
stm.execute(sql);
response.sendRedirect("login.jsp");
}
catch (Exception e)
{
e.printStackTrace();
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
}
}
<file_sep>import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.lang.*;
import javax.servlet.http.HttpSession;
import java.text.SimpleDateFormat;
import java.sql.Statement;
import java.sql.DriverManager;
@WebServlet(name = "AddComment")
public class AddComment extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String iid = request.getParameter("item_id");
String content = request.getParameter("content");
HttpSession session = request.getSession();
response.getWriter().write("item_id");
try {
Class.forName("com.mysql.jdbc.Driver");
java.util.Date d=new java.util.Date();
SimpleDateFormat sdf=new SimpleDateFormat("yyyy-MM-dd hh:mm:ss");
String da= sdf.format(d);//collect_time
Statement stm = DriverManager.getConnection("jdbc:mysql://localhost:3306/peproject", "root", "admin").createStatement();
String sql = "insert into peproject.comment(item_id,content,user_id,sender_id,time) \n" +
"values('"+ iid+"','"+ content +"','" + session.getAttribute("user_id") +"','"+iid+"','"+da+"')";
response.getWriter().println(sql);
stm.execute(sql);
response.sendRedirect("item_information.jsp?item_id="+iid);
} catch (Exception e) {
e.printStackTrace();
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
return;
}
}
<file_sep>
const banner = document.getElementById('banner-img');
const imgs = banner.getElementsByTagName('img');
console.log(imgs);
//全局变量,用于记录当前页面的值
var current = 0;
function changeImg(){
console.log(current);
// 将除了自己以外的全部设为none
for(let i=0;i<3;i++){
if (i!=current) {
imgs[i].style.display="none";
}
}
//全部消除了之后再设置
imgs[current].style.display="block"
}
//存放interval的id
var result ;
console.log('hello');
// 添加hover事件,取消轮播
banner.addEventListener('mouseover',()=>{
console.log('mouseover');
clearInterval(result);
},true);
banner.addEventListener('mouseout',()=>{
//定时轮播
result= setInterval(()=>{
// 超过2之后要从头开始
if (current==3) {
current=0;
}
//console.log(current);
changeImg();
changeDot(current);
current++;
},1000);
},true);
var dots = document.getElementById('dots').getElementsByTagName('span');
function changeDot(index){
for(let j=0;j<3;j++){
dots[j].className = 'dot-native';
}
dots[index].className = 'dot-active';
}
for(let i=0;i<3;i++){
let temp = dots[i];
temp.addEventListener('click',()=>{
changeDot(i);
current=i;
changeImg();
});
}
//定时轮播
result= setInterval(()=>{
if (current==3) {
current=0;
}
changeImg();
changeDot(current);
current++;
},1000);
// All_item
function tabPageControl(n)
{
for (var i = 0; i < tabTitles.cells.length; i++)
{
tabTitles.cells[i].className = "tabTitleUnSelected";
}
tabTitles.cells[n].className = "tabTitleSelected";
for (var i = 0; i < tabPagesContainer.tBodies.length; i++)
{
tabPagesContainer.tBodies[i].className = "tabPageUnSelected";
}
tabPagesContainer.tBodies[n].className = "tabPageSelected";
}
//var files = document.getElementById('pic').files;
var form = new FormData();//通过HTML表单创建FormData对象
var url = '127.0.0.1:8080/'
function selectFile(){
var files = document.getElementById('pic').files;
console.log(files[0]);
if(files.length == 0){
return;
}
var file = files[0];
//把上传的图片显示出来
var reader = new FileReader();
// 将文件以Data URL形式进行读入页面
console.log(reader);
reader.readAsBinaryString(file);
reader.onload = function(f){
var result = document.getElementById("result");
var src = "data:" + file.type + ";base64," + window.btoa(this.result);
result.innerHTML = '<img src ="'+src+'"/>';
}
console.log('file',file);
form.append('file',file);
console.log(form.get('file'));
}
| 3bb653e00241bd7eaa17553d4f5f08ee78dbff60 | [
"JavaScript",
"Java",
"INI"
] | 4 | INI | AlbertZENGcn/PE_Project | a00ca49ec6e30252db29c270666477e8a2dd83de | 1671d65d2419c401022dfadf4673939ed84f4764 |
refs/heads/master | <repo_name>vedank/MySampleWinApp<file_sep>/MySampleWinApp/Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace MySampleWinApp
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
var resp = CalulateLogicTC1(2,5);
label1.Text = "Done - " + resp;
}
public int CalulateLogicTC1(int a, int b)
{
return (a + b) * a;
}
private void button2_Click(object sender, EventArgs e)
{
var resp = CalulateLogicTC2(2, 5);
label1.Text = "OK Done - " + resp;
}
public int CalulateLogicTC2(int a, int b)
{
return a + b * a ;
}
}
}
<file_sep>/README.md
# MySampleWinApp
Its a sample window application - testing purpose for continuous integartion.
<file_sep>/WinAppUnitTest/TestSampleApp.cs
using System;
using NUnit.Framework;
using MySampleWinApp;
namespace WinAppUnitTest
{
[TestFixture]
public class TestSampleApp
{
[Test]
public void CalulateLogicTC1_Input2And5_14()
{
Form1 frm = new Form1();
var result = frm.CalulateLogicTC1(2, 5);
Assert.That(result,Is.EqualTo(14));
}
[Test]
public void CalulateLogicTC2_Input2And5_12()
{
Form1 frm = new Form1();
var result = frm.CalulateLogicTC2(2, 5);
Assert.That(result, Is.EqualTo(12));
}
}
}
| c7acdb464fbba91ecc326c9cd0b7a2f4f291b4ee | [
"Markdown",
"C#"
] | 3 | C# | vedank/MySampleWinApp | 57f3855f0fd0c5fce394c43d959cc98f6f5c3cf6 | ada58b50c6c761a4878aee8c56d65b70370f6181 |
refs/heads/master | <file_sep><?php
namespace App\Http\Controllers;
use App\Models\Produk;
use Illuminate\Http\Request;
class ProdukController extends Controller
{
public function create(Request $request)
{
$judulIklan = $request->input('judulIklan');
$danaNeed = $request->input('danaNeed');
$danaCollected = $request->input('danaCollected');
$url = $request->input('url');
$cerita = $request->input('cerita');
$produk = Produk::create([
'judulIklan' => $judulIklan,
'danaNeed' => $danaNeed,
'danaCollected' => $danaCollected,
'url'=>$url,
'cerita'=>$cerita
]);
return $this->responseHasil(200, true, $produk);
}
public function list()
{
$produk = Produk::all();
return $this->responseHasil(200, true, $produk);
}
public function show($id)
{
$produk = Produk::findOrFail($id);
return $this->responseHasil(200, true, $produk);
}
public function update(Request $request, $id)
{
$judulIklan = $request->input('judulIklan');
$danaNeed = $request->input('danaNeed');
$danaCollected = $request->input('danaCollected');
$url = $request->input('url');
$cerita = $request->input('cerita');
$produk = Produk::findOrFail($id);
$result = $produk->update([
'judulIklan' => $judulIklan,
'danaNeed' => $danaNeed,
'danaCollected' => $danaCollected,
'url'=>$url,
'cerita'=>$cerita
]);
return $this->responseHasil(200, true, $result);
}
public function delete($id)
{
$produk = Produk::findOrFail($id);
$delete = $produk->delete();
return $this->responseHasil(200, true, $delete);
}
}
<file_sep><?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Produk extends Model
{
protected $table = 'produk';
protected $fillable = ['judulIklan','danaNeed','danaCollected','url','cerita'];
public $timestamps = false;
}
<file_sep>-- phpMyAdmin SQL Dump
-- version 5.0.2
-- https://www.phpmyadmin.net/
--
-- Host: 127.0.0.1
-- Generation Time: Jun 24, 2021 at 05:25 PM
-- Server version: 10.4.14-MariaDB
-- PHP Version: 7.4.10
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
START TRANSACTION;
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- Database: `donasi_api`
--
-- --------------------------------------------------------
--
-- Table structure for table `member`
--
CREATE TABLE `member` (
`id` int(11) NOT NULL,
`nama` varchar(255) NOT NULL,
`email` varchar(255) NOT NULL,
`password` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Dumping data for table `member`
--
INSERT INTO `member` (`id`, `nama`, `email`, `password`) VALUES
(1, 'adminis', '<EMAIL>', <PASSWORD>'),
(2, 'admin', '<EMAIL>', <PASSWORD>'),
(3, 'asd', '<EMAIL>', <PASSWORD>'),
(4, 'fga', '<EMAIL>', <PASSWORD>'),
(5, 'ssss', '<EMAIL>', <PASSWORD>');
-- --------------------------------------------------------
--
-- Table structure for table `member_token`
--
CREATE TABLE `member_token` (
`id` int(11) NOT NULL,
`member_id` int(11) NOT NULL,
`auth_key` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Dumping data for table `member_token`
--
INSERT INTO `member_token` (`id`, `member_id`, `auth_key`) VALUES
(1, 1, '<KEY>'),
(2, 2, '<KEY>'),
(3, 3, '<KEY>'),
(4, 4, '<KEY>PozCaH8dKsnjEMc70ElJM1n1AWKV5rqwsn6d7UFrqsOB9msWjEvwEGWF7'),
(18, 5, 'LGZfDs7r3FBNPA7CCtLs23U3gVB9YlmdKvthAlOhY15XstjcJ9f7Bidi8Q41FqVyA2omusPjmCJlhosqJDGRrOBoAK9FanH1KIqJ');
-- --------------------------------------------------------
--
-- Table structure for table `produk`
--
CREATE TABLE `produk` (
`id` int(11) NOT NULL,
`judul_iklan` varchar(255) NOT NULL,
`dana_need` int(128) NOT NULL,
`dana_collected` int(128) NOT NULL,
`url` varchar(512) NOT NULL,
`cerita` varchar(512) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Dumping data for table `produk`
--
INSERT INTO `produk` (`id`, `judul_iklan`, `dana_need`, `dana_collected`, `url`, `cerita`) VALUES
(4, 'RS Apung Karam: Bangun Kembali Kapal RSA dr Lie II', 500000000, 3004560, 'https://media-cdn.tripadvisor.com/media/photo-s/0d/7c/59/70/farmhouse-lembang.jpg', 'Musibah telah menimpa Rumah Sakit Apung (RSA) dr. Lie Dharmawan hingga karam di sekitar perairan Selat Sape, Nusa Tenggara Barat pada hari Rabu (16/6) kemarin.\r\nSetelah menyelesaikan pelayanan medis di Pulau Semau, Kupang, Nusa Tenggara Timur (14/6), kapal berlayar dari Pelabuhan Tenau, Kupang menuju Torano, Sumbawa Besar, Nusa Tenggara Barat, tempat pelayanan medis berikutnya. \r\n\r\nNamun sebelum sampai ke pelabuhan, kapal karam di sekitar perairan Selat Sape, Nusa Tenggara Barat pada pukul 14.00 WIT.'),
(5, 'Bantu Anak Palestina Lewati Musim Dingin Mematikan', 300000000, 2500000, 'https://media-cdn.tripadvisor.com/media/photo-s/0d/7c/59/70/farmhouse-lembang.jpg', '51% warga Palestina menghadapi musim dingin, tanpa tempat tinggal yang memadai, dan persediaan makanan yang tak menentu mereka harus bisa bertahan hidup.\r\nMusim dingin kerap menjadi ancaman paling mematikan bagi penduduk di negara yang dirundung konflik. Pasalnya, penduduk di negara itu hidup dalam serba keterbatasan dalam menghadapi musim dingin, keterbatasan makanan, keterbatasan tempat tinggal, keterbatasan bahan bakar untuk pemanas ruangan atau pun selimut. '),
(6, 'Pusat Cahaya Islam di Adonara Barat Terancam Rubuh', 900000000, 45000000, 'https://media-cdn.tripadvisor.com/media/photo-s/0d/7c/59/70/farmhouse-lembang.jpg', 'Banjir bandang dan longsor yang menerjang NTT awal bulan April 2021 lalu, telah menyisakan duka mendalam. Rumah, fasilitas umum, hingga rumah ibadah sebagian besar telah rata dengan tanah. Warga kehilangan keluarga hingga harta benda. Luapan air dan lahar dingin ini tak pandang bulu, termasuk pada pesantren satu-satunya di Adonara Barat, NTT. '),
(9, 'Sedekah Jariyah 10.000 Quran ke Penjuru Negeri', 40000000, 2000000, 'https://media-cdn.tripadvisor.com/media/photo-s/0d/7c/59/70/farmhouse-lembang.jpg', '“Jika manusia mati, maka terputuslah amalnya kecuali tiga perkara: (1) sedekah jariyah, (2) ilmu yang diambil manfaatnya, (3) anak shalih yang selalu mendoakan orang tuanya.” (HR. Muslim, no. 1631)\r\n\r\nMelalui program Syiar Qur\'an, Rumah Zakat mengajak kalian untuk ikut menyalurkan 10,000 paket Al-Qur\'an dan Iqro ke wilayah korban bencana di seluruh Indonesia.\r\nQur\'an juga akan dibagikan untuk Desa Berdaya (binaan Rumah Zakat) dan atau pun Non Desa Berdaya.\r\n\r\nInsyaAllah Qur\'an ini dapat menjadi amal jariyah');
--
-- Indexes for dumped tables
--
--
-- Indexes for table `member`
--
ALTER TABLE `member`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `member_token`
--
ALTER TABLE `member_token`
ADD PRIMARY KEY (`id`),
ADD KEY `member_id` (`member_id`);
--
-- Indexes for table `produk`
--
ALTER TABLE `produk`
ADD PRIMARY KEY (`id`);
--
-- AUTO_INCREMENT for dumped tables
--
--
-- AUTO_INCREMENT for table `member`
--
ALTER TABLE `member`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=6;
--
-- AUTO_INCREMENT for table `member_token`
--
ALTER TABLE `member_token`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=19;
--
-- AUTO_INCREMENT for table `produk`
--
ALTER TABLE `produk`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=13;
--
-- Constraints for dumped tables
--
--
-- Constraints for table `member_token`
--
ALTER TABLE `member_token`
ADD CONSTRAINT `member_token_ibfk_1` FOREIGN KEY (`member_id`) REFERENCES `member` (`id`) ON DELETE NO ACTION ON UPDATE CASCADE;
COMMIT;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
| 0ef6d02b0ed25085787b56666468b5c44e4701e9 | [
"SQL",
"PHP"
] | 3 | PHP | SYZART/donasi-api | ffb607a9d5eb634516c7e407a91ab9d1c4424df4 | 16ba74ef73b42efd2b23bbdb5f33ad252f1aae66 |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Android.App;
using Android.OS;
using Android.Runtime;
using Android.Support.Design.Widget;
using Android.Support.V7.App;
using Android.Views;
using Android.Widget;
using Plugin.Permissions;
using Plugin.Permissions.Abstractions;
using Permission = Plugin.Permissions.Abstractions.Permission;
namespace PermissionSample.Droid
{
[Activity(Label = "@string/app_name", Theme = "@style/AppTheme.NoActionBar", MainLauncher = true)]
public class MainActivity : AppCompatActivity
{
TextView _resultText;
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
SetContentView(Resource.Layout.activity_main);
Plugin.CurrentActivity.CrossCurrentActivity.Current.Init(this, savedInstanceState);
Android.Support.V7.Widget.Toolbar toolbar = FindViewById<Android.Support.V7.Widget.Toolbar>(Resource.Id.toolbar);
SetSupportActionBar(toolbar);
FloatingActionButton fab = FindViewById<FloatingActionButton>(Resource.Id.fab);
fab.Click += FabOnClick;
_resultText = FindViewById<TextView>(Resource.Id.ResultText);
var phoneButton = FindViewById<Button>(Resource.Id.PhoneButton);
phoneButton.Click += PhoneButton_Click;
var locationButton = FindViewById<Button>(Resource.Id.LocationButton);
locationButton.Click += LocationButton_Click;
var cameraButton = FindViewById<Button>(Resource.Id.CameraButton);
cameraButton.Click += CameraButton_Click;
}
private async void PhoneButton_Click(object sender, EventArgs e)
{
var status = await Core.PermissionManager.RequestPermissionsAsync(Permission.Phone, Permission.Contacts);
_resultText.Text = GetPermissionStatus(status);
}
private async void LocationButton_Click(object sender, EventArgs e)
{
var status = await Core.PermissionManager.RequestPermissionsAsync(Permission.Location);
_resultText.Text = GetPermissionStatus(status);
}
private async void CameraButton_Click(object sender, EventArgs e)
{
var status = await Core.PermissionManager.RequestPermissionsAsync(Permission.Camera, Permission.MediaLibrary);
_resultText.Text = GetPermissionStatus(status);
}
private string GetPermissionStatus(Dictionary<Permission, PermissionStatus> status)
{
var sb = new StringBuilder();
sb.Append("Result:\n\n");
foreach (var pair in status)
{
sb.Append($"Permission: {pair.Key.ToString()}\n" +
$"Status: {pair.Value.ToString()}\n\n");
}
return sb.ToString();
}
public override bool OnCreateOptionsMenu(IMenu menu)
{
MenuInflater.Inflate(Resource.Menu.menu_main, menu);
return true;
}
public override bool OnOptionsItemSelected(IMenuItem item)
{
int id = item.ItemId;
if (id == Resource.Id.action_settings)
{
return true;
}
return base.OnOptionsItemSelected(item);
}
private void FabOnClick(object sender, EventArgs eventArgs)
{
View view = (View) sender;
Snackbar.Make(view, "Replace with your own action", Snackbar.LengthLong)
.SetAction("Action", (Android.Views.View.IOnClickListener)null).Show();
}
public override void OnRequestPermissionsResult(int requestCode, string[] permissions, [GeneratedEnum] Android.Content.PM.Permission[] grantResults)
{
PermissionsImplementation.Current.OnRequestPermissionsResult(requestCode, permissions, grantResults);
base.OnRequestPermissionsResult(requestCode, permissions, grantResults);
}
}
}
<file_sep>using Plugin.Permissions;
using Plugin.Permissions.Abstractions;
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
namespace PermissionSample.Core
{
public static class PermissionManager
{
/// <summary>
/// PermissionPluginを使用してパーミッションをリクエストします。
/// </summary>
/// <param name="permissions"></param>
/// <returns>PermissionとPermissionStatusのDictionary</returns>
/// <remarks>https://github.com/jamesmontemagno/PermissionsPlugin</remarks>
public static async Task<Dictionary<Permission, PermissionStatus>> RequestPermissionsAsync(params Permission[] permissions)
{
var _permissionStatus = new Dictionary<Permission, PermissionStatus>();
foreach (var permission in permissions)
{
try
{
var status = await CrossPermissions.Current.CheckPermissionStatusAsync(permission);
if (status != PermissionStatus.Granted)
{
// どのパーミッションで根拠を示す必要があるのか不明
if (await CrossPermissions.Current.ShouldShowRequestPermissionRationaleAsync(permission))
{
// ダイアログを表示する必要があるので、リクエストより前に確認する必要がありそう。
}
// ここでパーミッション確認のダイアログが表示されます。
var results = await CrossPermissions.Current.RequestPermissionsAsync(permission);
//Best practice to always check that the key exists
if (results.ContainsKey(permission))
status = results[permission];
}
if (status == PermissionStatus.Granted)
{
_permissionStatus.Add(permission, status);
}
else
{
_permissionStatus.Add(permission, status);
}
}
catch (Exception ex)
{
System.Diagnostics.Debug.WriteLine(ex.Message);
_permissionStatus.Clear();
_permissionStatus.Add(permission, PermissionStatus.Unknown);
return _permissionStatus;
}
}
return _permissionStatus;
}
}
}
<file_sep># PermissionSample
Android permission sample
| f3eba5a4fe7a83a228bfc2725b23e691efbcf1c9 | [
"Markdown",
"C#"
] | 3 | C# | ytabuchi/PermissionSample | cac8909c22fc7597cb4aacd3cd2d97ae65a29acd | 6e177c3c9f1e7150905c8bd4b3aaae127629ff60 |
refs/heads/master | <file_sep>import os
import gzip
import numpy as np
import tensorflow as tf
import scipy, scipy.io, scipy.io.wavfile
import argparse
import pandas
from tqdm import tqdm
pandas.set_option('display.max_colwidth',1000)
# Some parameters
WAV_RATE = 22050
WAV_LENGHT = 220500
dl_dir = '../download/'
# Parse the arguments
parser = argparse.ArgumentParser(description='build the tfwriter')
parser.add_argument('dataset', nargs='*', type=str,
default=['train'],
help='dataset to build (train or validate)')
args = parser.parse_args()
record_file_name = 'audioset_' + args.dataset[0] + '.tfrecords'
# Open the desired CSVs
# 1) Label indes and names
df_lbls_idx = pandas.read_csv(dl_dir + 'class_labels_indices.csv', quotechar='"')
# 2) Datsaset videos with start time, end time and labels
if args.dataset[0] == "train":
df_dataset = pandas.read_csv(dl_dir + 'balanced_train_segments.csv',
names=['f_id', 'start', 'end', 'lbls'],
quotechar='"',
skipinitialspace=True,
skiprows=2)
if args.dataset[0] == "validate":
df_dataset = pandas.read_csv(dl_dir + 'eval_segments.csv',
names=['f_id', 'start', 'end', 'lbls'],
quotechar='"',
skipinitialspace=True,
skiprows=3)
def _bytes_feature(value):
return tf.train.Feature(bytes_list=tf.train.BytesList(value=[value]))
def _int64_feature(values):
return tf.train.Feature(int64_list=tf.train.Int64List(value=values))
def _get_wav_lbls(wav_name):
# Retrieve the labels corresponding to file <wav_name>
labels = df_dataset[
df_dataset.f_id.str.contains(wav_name)] # find the labels
labels = labels.lbls.to_string(index=False) # /m/085jw,/m/0l14l2
labels = labels.split(',') # [u'/m/085jw', u'/m/0l14l2']
labels = map(str, labels)
return labels
def _get_lbl_idx(lbl):
# Retrieve index of given label
label_idx = df_lbls_idx[
df_lbls_idx.mid.str.contains(lbl)]
label_idx = int(label_idx['index'])
return label_idx
# Create a tfrecord file and list the wav's paths
writer = tf.python_io.TFRecordWriter(record_file_name)
wav_paths = [f for f in os.listdir(dl_dir + args.dataset[0] + '/') if '.wav.gz' in f]
for wav_path in tqdm(wav_paths):
# Read the gzipped wav files
wav_unzip = gzip.open(dl_dir + args.dataset[0] + '/' + wav_path)
wav = scipy.io.wavfile.read(wav_unzip)[1]
if len(wav.shape) > 1:
wav = wav.mean(axis=1)
wav_const = np.zeros((WAV_LENGHT), dtype='int16')
wav_const[:wav.shape[0]] = wav
# Retrieve the features to record
wav_name = wav_path[:11]
wav_labels = _get_wav_lbls(wav_name)
wav_labels = map(_get_lbl_idx, wav_labels)
wav_const = wav_const.tostring()
# Features in an array
feature={'raw': _bytes_feature(wav_const),
'labels': _int64_feature(wav_labels),
'name': _bytes_feature(wav_name) }
sample = tf.train.Example(features=tf.train.Features(feature=feature))
# Write to tfrecord
writer.write(sample.SerializeToString())
writer.close()<file_sep># audioset_raw
Download and create a tfreader for the audioset dataset
## Download dataset (takes few hours)
```bash
cd download/train
cat ../balanced_train_segments.csv | ../download.sh
cd ../validate
cat ../eval_segments.csv | ../download.sh
```
## Access the data (either through TFRecord or a Generator)
* ### Build the TFRecord file
```bash
cd download
python audioset_writer.py train
python audioset_writer.py validate
```
* ### Access WAV through a simple generator
``` python
from audioset_generator import get_batchs
# get_batchs is a generator
my_gen = get_batchs(batch_size=10, n_epochs=1, num_threads=4)
my_gen.next()
```
<file_sep>from Queue import Queue
from threading import Thread
import scipy.io.wavfile
import pandas as pd
import numpy as np
import random
import time
import gzip
import os
# Some parameters
N_CLASSES = 527
WAV_RATE = 22050
WAV_LENGHT = 220500
dl_dir = './download/'
ds_dir = dl_dir + 'train/'
label_indices = pd.read_csv(dl_dir + 'class_labels_indices.csv', quotechar='"')
label_indices = label_indices.values.tolist()
dataset = pd.read_csv(dl_dir + 'balanced_train_segments.csv',
names=['f_id', 'start', 'end', 'lbls'],
quotechar='"', skiprows=2, skipinitialspace=True)
dataset = dataset.values.tolist()
def _get_wav_lbls(wav_name):
# Retrieve the labels corresponding to file <wav_name>
labels = [item[3] for item in dataset if item[0] == wav_name][0]
labels = labels.split(',') # [u'/m/085jw', u'/m/0l14l2']
labels = map(str, labels)
return labels
def _get_lbl_idx(lbl):
# Retrieve index of given label
label_idx = [item[0] for item in label_indices if item[1] == lbl][0]
return label_idx
def _get_sample(wav_path):
# Extract WAV's name
wav_name = wav_path.split('/')[-1][:11]
# Open the wav and retrieve its labels
wav = gzip.open(wav_path)
wav = scipy.io.wavfile.read(wav)[1]
if len(wav.shape) > 1:
wav = wav.mean(axis=1)
wav_const = np.zeros((WAV_LENGHT), dtype='int16')
wav_const[:wav.shape[0]] = wav
# Acess WAV's labels
wav_lbls_idx = _get_wav_lbls(wav_name)
wav_lbls_idx = map(_get_lbl_idx, wav_lbls_idx)
wav_lbls_dense = np.zeros((N_CLASSES))
wav_lbls_dense[wav_lbls_idx] = 1
return wav_const, wav_lbls_dense
def fill_batch_queue(paths_q, batch_q, batch_size):
while paths_q.qsize() > batch_size:
# Create the batch of <batch_size> samples
wav_batch = np.ndarray((batch_size, WAV_LENGHT))
lbls_batch = np.ndarray((batch_size, N_CLASSES))
for idx in range(batch_size):
wav_path = paths_q.get()
paths_q.task_done()
wav, lbls = _get_sample(wav_path)
wav_batch[idx,:] = wav
lbls_batch[idx,:] = lbls
# Put the batch in another queue
batch_q.put((wav_batch, lbls_batch))
def _get_queue_from_dir_path(dataset_dir):
# Read wav_paths and put them into a queue
paths_q = Queue(maxsize=0)
wav_paths = [ds_dir + f for f in os.listdir(ds_dir) if '.wav.gz' in f]
random.shuffle(wav_paths)
for item in wav_paths:
paths_q.put(item)
return paths_q
def get_batchs(batch_size=10, n_epochs=1, num_threads=4):
for _ in range(n_epochs):
paths_q = _get_queue_from_dir_path(ds_dir)
batch_q = Queue(maxsize=num_threads*2)
# Start the Threads :
# They'll stop when size of <paths_q> is less than a batch size.
for i in range(num_threads):
worker = Thread(target=fill_batch_queue,
args=(paths_q, batch_q, batch_size))
worker.setDaemon(True)
worker.start()
while paths_q.qsize() > batch_size:
if batch_q.empty() == False:
wav_batch, lbls_batch = batch_q.get()
batch_q.task_done()
print lbls_batch[0][:10]
yield wav_batch, lbls_batch
get_batchs()
<file_sep>import tensorflow as tf
import matplotlib.pyplot as plt
import scipy, scipy.io, scipy.io.wavfile
import sounddevice as sd
import numpy as np
import pandas
import time
# Some parameters
WAV_RATE = 22050
WAV_LENGHT = 220500
N_CLASSES = 527
dl_dir = '../download/'
df_lbls_idx = pandas.read_csv(dl_dir + 'class_labels_indices.csv',
quotechar='"')
df_lbls_idx = np.array(df_lbls_idx)
def _get_label_names(dense_labels):
indexes = np.argwhere(dense_labels).reshape(-1)
indexes = list(indexes)
indexes = df_lbls_idx[indexes, 2]
indexes = ", ".join(indexes)
return indexes
def read_and_decode(filename_queue):
"""Read and decode my tf_record file containing the raw audioset
"""
reader = tf.TFRecordReader()
_, serialized_example = reader.read(filename_queue)
# Features expected on the tf_record
features = tf.parse_single_example(
serialized_example,
features={'raw': tf.FixedLenFeature([], tf.string),
'labels': tf.VarLenFeature(tf.int64),
'name': tf.FixedLenFeature([], tf.string) })
# Read and decode raw audio
wav_raw = tf.decode_raw(features['raw'], tf.int16)
wav_raw = tf.cast(wav_raw, tf.float64) * (1./30000)
wav_raw = tf.reshape(wav_raw, [WAV_LENGHT])
# Read and decode labels and name
wav_labels = features['labels']
wav_labels = tf.sparse_to_dense(features["labels"].values,
(N_CLASSES,), 1,
validate_indices=False)
wav_labels = tf.cast(wav_labels, tf.bool)
wav_name = features['name']
return wav_raw, wav_labels
def inputs(tfrecords_filename, batch_size=30, num_epochs=None):
"""Reads input data num_epochs times.
Args:
dataset: select between 'train', 'valid' and 'test'
batch_size: Number of examples per returned batch.
num_epochs: Number of times to read the input data, or 0/None to
train forever.
Returns:
A tuple (images, labels), where:
* wavs is a float tensor with shape [batch_size, WAV_LENGHT]
in the range [-1, 1].
* labels is an int32 tensor with shape [batch_size] with the true label,
a number in the range [0, mnist.NUM_CLASSES).
Note that an tf.train.QueueRunner is added to the graph, which
must be run using e.g. tf.train.start_queue_runners().
"""
with tf.name_scope('input'):
filename_queue = tf.train.string_input_producer(
[tfrecords_filename], num_epochs=num_epochs)
# Even when reading in multiple threads, share the filename queue.
wav, lbls = read_and_decode(filename_queue)
# Shuffle the examples and collect them into <batch_size> batches.
# (Internally uses a RandomShuffleQueue.)
# We run this in two threads to avoid being a bottleneck.
wavs, sparse_labels = tf.train.shuffle_batch(
[wav, lbls],
batch_size=batch_size,
num_threads=2,
capacity=batch_size * 6,
min_after_dequeue=batch_size * 3)
return wavs, sparse_labels
"""
Here begins the desired shit ;)
"""
def main():
waves, labels = inputs(dl_dir + 'audioset_train.tfrecords', batch_size=3)
init_op = tf.group(tf.global_variables_initializer(),
tf.local_variables_initializer())
with tf.Session() as sess:
sess.run(init_op)
# Start batch threads
coord = tf.train.Coordinator()
threads = tf.train.start_queue_runners(sess=sess, coord=coord)
m_waves, m_labels = sess.run([waves, labels])
for wav, lbl in zip(m_waves, m_labels):
print _get_label_names(lbl)
sd.play(wav, WAV_RATE)
time.sleep(10)
sd.stop()
coord.request_stop()
coord.join(threads)
if __name__ == '__main__':
main() | f5963b78f4648a8011d3dcb3af697c16e310d160 | [
"Markdown",
"Python"
] | 4 | Python | marc-moreaux/audioset_raw | 36e18a3160245b3d8873667d25f719e2084e3be7 | 2dd509e3acbde8ec1a3a0c7f63f4234c2474a5d5 |
refs/heads/master | <file_sep>(function($){
$(document).ready(function (){
$('.of-iconmntop').click(function(){
$('.of-menutop').toggleClass('of-mnshow');
});
$('.of-search').click(function(){
$('.of-searchbox').show(200);
});
$('.of-closesearch').click(function(){
$('.of-searchbox').hide(200);
});
$(document).on('click','[toscroll]',function(e){
e.preventDefault();
var link = $(this).attr('toscroll');
if($(link).length > 0){
var posi = $(link).offset().top - 50;
$('body,html').animate({scrollTop:posi},1000);
}
});
$(window).bind('scroll', function () {
if ($(window).scrollTop() > 500) {
$('.mi-menu').addClass('mi-mnfix');
} else {
$('.mi-menu').removeClass('mi-mnfix');
}
});
/*10042018*/
$(document).on('click','.xs-verbtn a',function () {
var txtpro = $(this).attr("data-prod");
$('.xs-verul li').removeClass('active');
$('.tabs2').addClass('active');
$('.tabs1 a').text(txtpro);
});
$(document).on('click','.tabstab ul li',function () {
$('.xs-verul li').removeClass('active');
$('.tabs3').addClass('active');
var txtcolor = $(this).attr("data-color");
$('.tabs2 a').text(txtcolor);
});
/*08102018*/
$('#xsvdmd').on('show.bs.modal', function () {
$('.ac-video-media-controller')[0].play();
}).on('hide.bs.modal', function () {
$('.ac-video-media-controller')[0].pause();
});
});
})(window.jQuery);<file_sep>(function($){
var slickNews = {
init: function () {
slickNews.events();
},
events: function () {
$('.of9-s2wrap').countdown('2018/08/15 00:00', function(event) {
$(this).html(event.strftime('<span class="glyphicon glyphicon-time of9-s2icon"></span> <span class="of9-s2tit">Oppo F9 sẽ ra mắt sau</span> <i></i> <span class="of9-s2time of9-s2tm1">%D</span> <span class="of9-s2tit">ngày</span> <span class="of9-s2time of9-s2tm2">%H:%M:%S</span>'));
});
}
};
$(document).ready(function (){
$('.of-iconmntop').click(function(){
$('.of-menutop').toggleClass('of-mnshow');
});
$('.of-search').click(function(){
$('.of-searchbox').show(200);
});
$('.of-closesearch').click(function(){
$('.of-searchbox').hide(200);
});
//landing
if ($(".of9-s2wrap").length > 0) {
slickNews.init();
}
wow = new WOW({
animateClass: 'animated',
offset: 5
});
wow.init();
$(document).on('click','[toscroll]',function(e){
e.preventDefault();
var link = $(this).attr('toscroll');
if($(link).length > 0){
var posi = $(link).offset().top - 50;
$('body,html').animate({scrollTop:posi},1000);
}
});
$(window).bind('scroll', function () {
if ($(window).scrollTop() > 70) {
$('.of9-menu').addClass('of9-mnfix');
} else {
$('.of9-menu').removeClass('of9-mnfix');
}
});
var swiper = new Swiper('.swiper-container', {
slidesPerView: 4,
spaceBetween: 20,
pagination: {
el: '.swiper-pagination',
clickable: true,
},
breakpoints: {
768: {
slidesPerView: 3,
spaceBetween: 10,
},
640: {
slidesPerView: 2,
spaceBetween: 5,
},
374: {
slidesPerView: 1,
spaceBetween: 0,
}
}
});
$(document).on('click','.of9-s4ldo',function () {
$('.of9-s4mau').hide();
$('.of9-s4do').show();
});
$(document).on('click','.of9-s4lxanh',function () {
$('.of9-s4mau').hide();
$('.of9-s4xanh').show();
});
/*09082018*/
$(document).on('click','.of9-vd2 span',function (ev) {
$(".of9-vdplay").slideDown();
$(".of9-video").slideUp();
$(".of9-vdplay iframe")[0].src += "&autoplay=1";
ev.preventDefault();
});
});
})(window.jQuery);<file_sep><!DOCTYPE html>
<html lang="en">
<head>
<title>FPT Shop</title>
<meta name="description" content="FPT Shop">
<meta name="keywords" content="FPTSHOP" />
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0">
<meta name="copyright" content="Công ty Cổ phần Bán lẻ Kỹ thuật số FPT">
<meta name="author" content="Công ty Cổ phần Bán lẻ Kỹ thuật số FPT">
<meta http-equiv="audience" content="General">
<meta name="resource-type" content="Document">
<meta name="distribution" content="Global">
<meta name="GENERATOR" content="Công ty Cổ phần Bán lẻ Kỹ thuật số FPT">
<meta property="fb:app_id" content="289591837916420" />
<meta property="article:publisher" content="https://www.facebook.com/FPTShopOnline" />
<meta property="article:author" content="https://www.facebook.com/FPTShopOnline" />
<meta property="og:image" content="http://fptshop.com.vn/Content/v3/images/logo-black.png" />
<meta property="og:title" content="FPTShop" />
<meta property="og:description" content="FPTShop" />
<link href="https://fonts.googleapis.com/css?family=Roboto:400,500,500i,700&subset=vietnamese" rel="stylesheet">
<!-- Favicons -->
<link rel="icon" href="images/favicon.ico">
<!-- Css -->
<link rel="stylesheet" href="css/bootstrap.min.css" />
<link rel="stylesheet" href="css/jquery.mCustomScrollbar.css" />
<link rel="stylesheet" href="css/swiper.min.css" />
<link rel="stylesheet" href="css/treset.css" />
<link rel="stylesheet" href="css/style.css" />
<!-- Js -->
<script src="js/libs/jquery-1.11.2.min.js"></script>
<script src="js/libs/bootstrap.min.js"></script>
<script src="js/libs/jquery.mCustomScrollbar.concat.min.js"></script>
<script src="js/libs/swiper.min.js"></script>
<script src="js/functions.js"></script>
<!-- HTML5 shim and Respond.js for IE8 support of HTML5 elements and media queries -->
<script src="js/libs/ie-emulation-modes-warning.js"></script>
<!--[if lt IE 9]>
<script src="js/libs/html5shiv.min.js"></script>
<script src="js/libs/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="of-header">
<div class="container">
<a href="http://fptshop.com.vn/" title="Fptshop.com.vn"><img class="of-logo" src="images/logo.png" alt="Fptshop.com.vn"></a>
<span class="of-iconmntop"><i class="glyphicon glyphicon-menu-hamburger"></i></span>
<ul class="of-menutop clearfix">
<li><a target="_blank" href="http://fptshop.com.vn/dien-thoai" title=""> <i class="of-imobile"></i> <span>Điện thoại</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/may-tinh-xach-tay" title=""> <i class="of-ilaptop"></i> <span>Laptop</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/apple" title=""> <i class="of-iapple"></i> <span>Apple</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/samsung" title="" > <i class="of-samsung"></i> <span>Samsung</span></a></li>
<li><a target="_blank" href="http://fptshop.com.vn/may-tinh-bang" title=""> <i class="of-itablet"></i> <span>Tablet</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/phu-kien" title=""> <i class="of-ipkien"></i> <span>Phụ kiện</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/may-doi-tra" title=""> <i class="of-imdt"></i> <span>Máy đổi trả</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/sim-the" title=""> <i class="of-isimthe"></i> <span>Sim thẻ</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/khuyen-mai" title=""> <i class="of-ikmai"></i> <span>Khuyến mãi</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/tin-tuc" title=""> <i class="of-itinhay"></i> <span>Tin hay</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/dien-dan" title=""> <i class="of-ihoidap"></i> <span>Hỏi đáp</span> </a></li>
</ul>
<div class="of-search">
<i class="of-isearch"></i> <span>Tìm kiếm</span>
</div>
<div class="of-call">
<a href="tel:18006601">
<span>Tư vấn miễn phí</span>
<p><i class="glyphicon glyphicon-earphone"></i>1800 6601</p>
</a>
</div>
</div>
</div>
<div class="of-searchbox">
<div class="of-searchbar">
<div class="container">
<a href="" title=""><img class="of-slogo" src="images/logo-mb.png" alt=""></a>
<div class="of-ipsearch input-group">
<span class="input-group-btn">
<button class="btn btn-default" type="button"><i class="glyphicon glyphicon-search"></i></button>
</span>
<input type="text" class="form-control" placeholder="Tìm kiếm Fptshop.com.vn">
</div>
<span class="of-closesearch">✕</span>
</div>
</div>
</div>
<div class="y9-main">
<div class="y9-s1">
<div class="row">
<div class="col-md-5 col-md-push-7">
<div class="y9-s1w">
<p><img src="images/0410/title-km.png" alt=""></p>
<p class="y9-btn"><a href="#" title="">ĐẶT TRƯỚC NGAY</a></p>
<p class="y9-s1ds">Đã có <strong>6969</strong> khách đặt hàng <span>Xem danh sách</span></p>
</div>
</div>
<div class="col-md-7 col-md-pull-5">
<p class="y9-s1i1"><img src="images/0410/s1.png" alt=""></p>
</div>
</div>
</div>
<div class="y9-menu">
<div class="container">
<h1 class="y9-logo"><img src="images/0410/lg-mn.png" alt=""></h1>
<ul class="y9-mnul clearfix">
<li><a toscroll="#dacdiem" href="javascript:void(0);" title="">ĐẶC ĐIỂM NỔI BẬT</a></li>
<li><a toscroll="#thongso" href="javascript:void(0);" title="">THÔNG SỐ KỸ THUẬT</a></li>
<li><a toscroll="#thele" href="javascript:void(0);" title="">THỂ LỆ</a></li>
<li><a toscroll="#binhluan" href="javascript:void(0);" title="">BÌNH LUẬN</a></li>
</ul>
<a class="y9-mnbtn" toscroll="#dathang" href="javascript:void(0);" title="">ĐẶT TRƯỚC</a>
</div>
</div>
<div class="y9-s2">
<div class="container">
<div class="y9-s2tabs">
<div class="y9-s2it">
<p class="y9-tit">GPU TURBO <span>Hiệu Năng Siêu Việt Cho Cuộc Vui Bất Tận</span></p>
<p class="y9-sub">So với thế hệ trước, chip Kirin 710 với 8-nhân cải thiện hiệu năng 75% so với 1-nhân và 68% so với hiệu đa-nhân. Tái định nghĩa khái niệm chơi game với GPU Turbo, tăng gấp đôi hiệu suất & gấp 1.3 lần hiệu năng, cho bạn đắm chìm trong trải nghiệm chơi game mượt mà đầy cảm xúc. </p>
</div>
<div class="y9-s2it">
<p class="y9-s2i"><img src="images/0410/s2.png" alt=""></p>
</div>
</div>
</div>
</div>
<div class="y9-s3">
<div class="y9-s3w">
<p class="y9-tit">Giải trí cực đã trên màn hình 6,5 inch siêu sắc nét</p>
<p class="y9-sub">Màn hình Huawei Y9 2019 có kích thước lên tới 6,5 inch độ phân giải Full HD+ siêu sắc nét, mang đến cho bạn những hình ảnh cực đã. Mọi thứ còn tuyệt vời hơn khi màn hình này thiết kế theo dạng viền siêu mỏng, cho cảm giác “tràn viền” đầy ấn tượng.</p>
</div>
<p class="y9-s3bg"><img src="images/0410/bg3.jpg" alt=""></p>
<p class="y9-s3bgm"><img src="images/0410/bg3-mb.png" alt=""></p>
</div>
<div class="y9-s4">
<div class="container">
<div class="y9-s4w">
<p class="y9-tit">Viên pin <span>bền bỉ 4000 mAh*</span></p>
<p class="y9-sub">Không chỉ mang trên mình viên pin dung lượng lớn 4000 mAh*, Huawei Y9 2019 còn có con chip Kirin 710 sản xuất trên tiến trình mới 12nm tiết kiệm điện. Bạn có thể sử dụng máy thoải mái cả ngày mà không lo hết pin ở tốc độ vô cùng mượt mà.</p>
<p class="y9-s3d">* Giá trị tiêu chuẩn</p>
</div>
<p class="y9-s4i"><img src="images/0410/s4.png" alt=""></p>
</div>
</div>
<div class="y9-s5">
<div class="y9-s5w">
<p class="y9-tit">Giải trí cực đã trên màn hình 6,5 inch siêu sắc nét</p>
<p class="y9-sub">Màn hình Huawei Y9 2019 có kích thước lên tới 6,5 inch độ phân giải Full HD+ siêu sắc nét, mang đến cho bạn những hình ảnh cực đã. Mọi thứ còn tuyệt vời hơn khi màn hình này thiết kế theo dạng viền siêu mỏng, cho cảm giác “tràn viền” đầy ấn tượng.</p>
</div>
<p class="y9-s5bg"><img src="images/0410/bg5.jpg" alt=""></p>
<p class="y9-s5bgm"><img src="images/0410/bg5-mb.png" alt=""></p>
</div>
<div class="y9-s6">
<div class="y9-s6w">
<p class="y9-tit">Bắt trọn cả thế giới nhờ 4 camera AI</p>
<p class="y9-sub">Sở hữu tới 4 camera được hỗ trợ bởi trí tuệ nhân tạo AI, Huawei Y9 2019 có khả năng chụp ảnh chuyên nghiệp một cách dễ dàng khi AI sẽ tự động nhận diện cảnh và tối ưu hợp lý, mang lại hình ảnh đẹp nhất. Hai cụm camera kép giúp bạn chụp chân dung xóa phông tuyệt đẹp bằng cả camera trước và sau.</p>
</div>
<p class="y9-s6i1"><img src="images/0410/icons6.png" alt=""></p>
<p class="y9-s6i1m"><img src="images/0410/icons6mb.png" alt=""></p>
<p class="y9-s6i2"><img src="images/0410/s6.png" alt=""></p>
</div>
<div id="hinhanh" class="y9-s7">
<div class="container">
<div class="row">
<div class="col-md-5">
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide y9-sd"><img src="images/0410/product-7.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/product-7.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/product-7.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/product-7.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/product-7.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/product-7.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/product-7.jpg" alt=""></div>
</div>
<!-- Add Pagination -->
<div class="swiper-pagination"></div>
</div>
<p class="y9-s7pr">Giá: <span>5.490.000đ</span> <a href="#" title="">Đặt trước ngay</a></p>
</div>
<div class="col-md-7">
<h2 class="nk6-s6hd">Thông số kỹ thuật</h2>
<ul class="nk6-s6ts">
<li><label>Màn hình:</label> <span>5.2" IPS LCD , 720 x 1280 pixels</span></li>
<li><label>Thiết kế:</label> <span>Nhựa nguyên khối</span></li>
<li><label>Camera sau:</label> <span>16MP + 5MP</span></li>
<li><label>Camera trước:</label> <span>16MP</span></li>
<li><label>Tính năng camera:</label> <span>Chụp ảnh siêu độ phân giải, Chế độ Time-Lapse, Tự động lấy nét, Chạm lấy nét, Nhận diện khuôn mặt, HDR, Panorama, PixelMaster, Ảnh GIF, Chế độ chụp chuyên nghiệp</span></li>
<li><label>CPU:</label> <span>Qualcomm Snapdragon 425, 1.4 Ghz, 4 nhân</span></li>
<li><label>RAM:</label> <span>2 GB</span></li>
<li><label>Bộ nhớ lưu trữ:</label> <span>16 GB</span></li>
<li><label>Hỗ trợ thẻ nhớ:</label> <span>MicroSD lên tới 256 GB</span></li>
<li><label>Thẻ sim:</label> <span>2 Sim (Nano SIM)</span></li>
<li><label>Dung lượng pin:</label> <span>4100 mAh</span></li>
<li><label>Trọng lượng:</label> <span>156 g</span></li>
<li><label>Kết nối mạng:</label> <span>LTE</span></li>
<li><label>Bảo mật thông minh:</label> <span>Vân tay</span></li>
<li><label>Cảm biến:</label> <span>Mở khoá vân tay 1 chạm</span></li>
</ul>
</div>
</div>
</div>
</div>
<div class="y9-s8">
<div class="container">
<h2 class="nk6-s6tt">Tin tức sản phẩm</h2>
<div class="swiper-container swiper-s8">
<div class="swiper-wrapper">
<div class="swiper-slide y9-sd"><img src="images/0410/10.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/10.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/10.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/10.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/10.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/10.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/10.jpg" alt=""></div>
<div class="swiper-slide y9-sd"><img src="images/0410/10.jpg" alt=""></div>
</div>
<!-- Add Pagination -->
<div class="swiper-pagination"></div>
</div>
</div>
</div>
<div class="ss-tlds" id="thele">
<div class="container">
<div class="row">
<div class="col-md-6 col-sm-12">
<h3 class="p1-s4tit">Thể lệ chương trình</h3>
<div class="p1-s4ttle mCustomScrollbar">
<p><strong>Thời gian:</strong>Áp dụng từ 05/04/2016</p>
<p><strong>Ưu đãi:</strong> khách hàng mua Galaxy S7, S7 edge nhận được tới <strong>9 ưu đãi khủng</strong>:</p>
<ul>
<li><strong>1:</strong> Trả góp Home Credit 0% lãi suất, trả trước
40%,
kỳ hạn 6 tháng </li>
<li><strong>2:</strong> Tặng Sạc dự phòng nhanh Samsung 5200mAh
(không
áp dụng trả góp 0%)</li>
<li><strong>3:</strong> Sim Đại Phát 0888xxxxx8 (không áp dụng trả
góp
0%)</li>
<li><strong>4:</strong> 3 tháng cước 3G trị giá 210.000đ (không áp
dụng
trả góp 0%)</li>
<li><strong>5:</strong> Phiếu mua hàng 220.000đ áp dụng mua Sạc dự
phòng
6000mAh hoặc 10000mAh</li>
<li><strong>6:</strong> Gói chăm sóc đặc quyền: 1 đổi 1, hỗ trợ kỹ
thuật
tận nhà, Hotline VIP</li>
<li><strong>7:</strong> Gói ưu đãi đặc quyền: phòng chờ máy bay hạng
thương gia, cafe/xem phim miễn phí rạp CGV, gói game-pack trị giá
200USD...></li>
<li><strong>8:</strong> Cơ hội trúng thưởng 27 Galaxy S7</li>
<li><strong>9:</strong> Cơ hội trúng thưởng 300 Tivi Samsung 32”</li>
</ul>
<p><strong>Thời gian mở bán:</strong>Từ 18/03/2016</p>
<p><strong>Ưu đãi:</strong> khách hàng mua Galaxy S7, S7 edge chọn 1 trong 3 gói khuyến mãi sau:</p>
<ul>
<li><strong>KM1:</strong> Trả góp Home Credit 0% lãi suất, trả trước 40%, kỳ hạn 6 tháng</li>
<li><strong>KM2:</strong> Tặng Sạc dự phòng nhanh Samsung 5200mAh + Sim Đại Phát 088</li>
<li><strong>KM3:</strong> Trả góp 0% bằng thẻ tín dụng + Sạc dự phòng nhanh Samsung 5200mAh + Sim Đại Phát 088 (áp dụng từ 14h 22/03)</li>
</ul>
<p><strong>Ngoài 1 trong 3 gói khuyến mãi trên, khách hàng nhận thêm:</strong></p>
<ul>
<li><strong>Gói ưu đãi đặc quyền:</strong> phòng chờ máy bay hạng thương gia, cafe/xem phim miễn phí rạp CGV, gói game trị giá 200USD...
<li><strong>Gói chăm sóc đặc quyền:</strong> 1 đổi 1, hỗ trợ kỹ thuật tận nhà, Hotline VIP</li>
<li><strong>Cơ hội trúng thưởng 27 Galaxy S7</strong> dành cho kháchhàng mua hàng từ 01/03 - 30/04 (không áp dụng cho khách hàng đã trúng thưởng đợt 1).</li>
<li><strong>Khuyến mãi phụ kiện giảm tới 30%</strong> khi mua kèm</li>
</ul>
<p><strong>Lưu ý:</strong>Không áp dụng đối với đơn hàng đặt cọc từ 01/03 đến 17/03.</p>
<p><strong>Thời gian đặt cọc: </strong>01/03/2016 - 22h00 17/03/2016</p>
<p><strong>Ưu đãi: </strong></p>
<p><strong>- Từ 01/03 đến 12h00 03/03:</strong> khách hàng may mắn có cơ hội nhận được</p>
</div>
</div>
<div class="col-md-6 col-sm-12">
<h3 class="p1-s4tit">Có <strong>132</strong> người chơi tham gia</h3>
<ul class="p1-s4ulsh clearfix">
<li>
<div class="p1-s4search">
<input type="text" class="form-control" placeholder="Nhập số điện thoại để tìm kiếm">
</div>
</li>
<li>
<input type="checkbox" class="p1-s4rad" id="p1s4rad5">
<label for="p1s4rad5" class="p1-s4lb">Chỉ hiển thị người trúng giải</label>
</li>
</ul>
<div class="p1-s4table mCustomScrollbar">
<table class="table table-hover table-striped">
<thead>
<tr>
<td>STT</td>
<td>Họ tên</td>
<td>Điện thoại</td>
<td>Trạng thái</td>
</tr>
</thead>
<tbody>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
</div>
<footer class="of-footer">
<div class="container">
<div class="of-footer-link">
<a target="_blank" href="http://fptshop.com.vn/huong-dan/huong-dan-mua-hang" title="Hướng dẫn mua hàng">Hướng dẫn mua hàng</a> |
<a target="_blank" href="http://fptshop.com.vn/ho-tro/chinh-sach-tra-gop" title="Trả góp">Trả góp</a> |
<a target="_blank" href="http://fptshop.com.vn/ho-tro/chinh-sach-bao-hanh" title="Chính sách bảo hành">Chính sách bảo hành</a>
</div>
<p>Công Ty Cổ Phần Bán Lẻ Kỹ Thuật Số FPT - 261-263 Khánh Hội, P5, Q4, TP. Hồ Chí Minh.</p>
<p>Điện thoại: 028.73023456. Fax: 028.39435773. Email: <EMAIL></p>
</div>
</footer>
</body>
</html>
<file_sep>(function($){
$(document).ready(function (){
$('.of-iconmntop').click(function(){
$('.of-menutop').toggleClass('of-mnshow');
});
$('.of-search').click(function(){
$('.of-searchbox').show(200);
});
$('.of-closesearch').click(function(){
$('.of-searchbox').hide(200);
});
$(document).on('click','[toscroll]',function(e){
e.preventDefault();
var link = $(this).attr('toscroll');
if($(link).length > 0){
var posi = $(link).offset().top - 50;
$('body,html').animate({scrollTop:posi},1000);
}
});
/*screen gameplay*/
$('.btnmoqua').click( function () {
$('.buoc2').show();
$('.buoc1').hide();
});
$('.btntruot').click( function () {
$('.buoc5').show();
$('.buoc1').hide();
});
$('.btngame').click( function () {
$('.buoc1').show();
$('.buoc2,.buoc3,.buoc4,.buoc5').hide();
})
});
})(window.jQuery);<file_sep>(function($){
$(document).ready(function (){
$('.of-iconmntop').click(function(){
$('.of-menutop').toggleClass('of-mnshow');
});
$('.of-search').click(function(){
$('.of-searchbox').show(200);
});
$('.of-closesearch').click(function(){
$('.of-searchbox').hide(200);
});
//landing
// $('.aw-ul').countdown('2018/09/13 00:00', function(event) {
// $(this).html(event.strftime('<li><p class="aw-p1">%D</p><p class="aw-p2">Ngày</p></li><li><p class="aw-p1">%H</p><p class="aw-p2">Giờ</p> </li><li> <p class="aw-p1">%M</p> <p class="aw-p2">Phút</p> </li><li> <p class="aw-p1">%S</p> <p class="aw-p2">Giây</p> </li>'));
// });
if ($(".of9-s2wrap").length > 0) {
slickNews.init();
}
$(document).on('click','[toscroll]',function(e){
e.preventDefault();
var link = $(this).attr('toscroll');
if($(link).length > 0){
var posi = $(link).offset().top - 50;
$('body,html').animate({scrollTop:posi},1000);
}
});
$(window).bind('scroll', function () {
if ($(window).scrollTop() > 500) {
$('.mi-menu').addClass('mi-mnfix');
} else {
$('.mi-menu').removeClass('mi-mnfix');
}
});
var swiper = new Swiper('.swiper-container', {
slidesPerView: 4,
spaceBetween: 20,
pagination: {
el: '.swiper-pagination',
clickable: true,
},
breakpoints: {
768: {
slidesPerView: 3,
spaceBetween: 10,
},
640: {
slidesPerView: 2,
spaceBetween: 5,
},
374: {
slidesPerView: 1,
spaceBetween: 0,
}
}
});
});
})(window.jQuery);<file_sep><!DOCTYPE html>
<html lang="en">
<head>
<title>FPT Shop</title>
<meta name="description" content="FPT Shop">
<meta name="keywords" content="FPTSHOP" />
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0">
<meta name="copyright" content="Công ty Cổ phần Bán lẻ Kỹ thuật số FPT">
<meta name="author" content="Công ty Cổ phần Bán lẻ Kỹ thuật số FPT">
<meta http-equiv="audience" content="General">
<meta name="resource-type" content="Document">
<meta name="distribution" content="Global">
<meta name="GENERATOR" content="Công ty Cổ phần Bán lẻ Kỹ thuật số FPT">
<meta property="fb:app_id" content="289591837916420" />
<meta property="article:publisher" content="https://www.facebook.com/FPTShopOnline" />
<meta property="article:author" content="https://www.facebook.com/FPTShopOnline" />
<meta property="og:image" content="http://fptshop.com.vn/Content/v3/images/logo-black.png" />
<meta property="og:title" content="FPTShop" />
<meta property="og:description" content="FPTShop" />
<!-- Favicons -->
<link rel="icon" href="images/favicon.ico">
<!-- Css -->
<link rel="stylesheet" href="css/bootstrap.min.css" />
<link rel="stylesheet" href="css/jquery.mCustomScrollbar.css" />
<link rel="stylesheet" href="css/treset.css" />
<link rel="stylesheet" href="css/style.css" />
<!-- Js -->
<script src="js/libs/jquery-1.11.2.min.js"></script>
<script src="js/libs/bootstrap.min.js"></script>
<script src="js/libs/jquery.mCustomScrollbar.concat.min.js"></script>
<script src="js/functions.js"></script>
<!-- HTML5 shim and Respond.js for IE8 support of HTML5 elements and media queries -->
<script src="js/libs/ie-emulation-modes-warning.js"></script>
<!--[if lt IE 9]>
<script src="js/libs/html5shiv.min.js"></script>
<script src="js/libs/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="of-header">
<div class="container">
<a href="http://fptshop.com.vn/" title="Fptshop.com.vn"><img class="of-logo" src="images/logo.png" alt="Fptshop.com.vn"></a>
<span class="of-iconmntop"><i class="glyphicon glyphicon-menu-hamburger"></i></span>
<ul class="of-menutop clearfix">
<li><a target="_blank" href="http://fptshop.com.vn/dien-thoai" title=""> <i class="of-imobile"></i> <span>Điện thoại</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/may-tinh-xach-tay" title=""> <i class="of-ilaptop"></i> <span>Laptop</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/apple" title=""> <i class="of-iapple"></i> <span>Apple</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/samsung" title="" > <i class="of-samsung"></i> <span>Samsung</span></a></li>
<li><a target="_blank" href="http://fptshop.com.vn/may-tinh-bang" title=""> <i class="of-itablet"></i> <span>Tablet</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/phu-kien" title=""> <i class="of-ipkien"></i> <span>Phụ kiện</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/may-doi-tra" title=""> <i class="of-imdt"></i> <span>Máy đổi trả</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/sim-the" title=""> <i class="of-isimthe"></i> <span>Sim thẻ</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/khuyen-mai" title=""> <i class="of-ikmai"></i> <span>Khuyến mãi</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/tin-tuc" title=""> <i class="of-itinhay"></i> <span>Tin hay</span> </a></li>
<li><a target="_blank" href="http://fptshop.com.vn/dien-dan" title=""> <i class="of-ihoidap"></i> <span>Hỏi đáp</span> </a></li>
</ul>
<div class="of-search">
<i class="of-isearch"></i> <span>Tìm kiếm</span>
</div>
<div class="of-call">
<a href="tel:18006601">
<span>Tư vấn miễn phí</span>
<p><i class="glyphicon glyphicon-earphone"></i>1800 6601</p>
</a>
</div>
</div>
</div>
<div class="of-searchbox">
<div class="of-searchbar">
<div class="container">
<a href="" title=""><img class="of-slogo" src="images/logo-mb.png" alt=""></a>
<div class="of-ipsearch input-group">
<span class="input-group-btn">
<button class="btn btn-default" type="button"><i class="glyphicon glyphicon-search"></i></button>
</span>
<input type="text" class="form-control" placeholder="Tìm kiếm Fptshop.com.vn">
</div>
<span class="of-closesearch">✕</span>
</div>
</div>
</div>
<style>
.ip-tit1{
font-weight: 600;
font-size: 28px;
text-align: center;
color: #ffe5db;
padding-top: 60px;
}
.ip-tit1 span{
font-weight: 300;
}
.ip-tit2{
font-size: 42px;
font-weight: 600;
line-height: 1.38;
color: #ffe5db;
padding-top: 10px;
}
.ip-tit2 span{
font-weight: 900;
}
.ip-img1{
padding: 30px 0 15px 0;
}
.ip-btn1{
display: flex;
justify-content: space-between;
padding: 0 15px;
}
.ip-btn1:hover .ip-btna,
.ip-ulp2:hover .ip-btna{
color: #ffffff;
}
.ip-btna{
display: inline-block;
font-size: 17px;
text-align: center;
color: #ffe5db;
height: 40px;
line-height: 40px;
padding: 0 40px;
border: solid 1px #4a4a4a;
-webkit-border-radius: 4px;
-moz-border-radius: 4px;
border-radius: 4px;
background-color: #333333;
}
.mi-mns{
margin-right: 0px;
}
.ip-tit2m{
font-size: 38px;
font-weight: 600;
line-height: 1.26;
text-align: center;
color: #ffe5db;
}
.ip-ul1 li{
padding: 30px 0;
display: table;
width: 100%;
position: relative;
}
.ip-ul1 li:before {
content: "";
display: inline-block;
width: 200px;
height: 1px;
position: absolute;
top: auto;
right: 0;
left: 50%;
bottom: 0;
margin-left: -100px;
background: -moz-linear-gradient(0deg, rgba(0,0,0,0) 0%, rgba(210,182,148,1) 50%, rgba(0,0,0,0) 100%);
background: -webkit-gradient(linear, left top, right top, color-stop(0%, rgba(0,0,0,0)), color-stop(50%, rgba(210,182,148,1)), color-stop(100%, rgba(0,0,0,0)));
background: -webkit-linear-gradient(0deg, rgba(0,0,0,0) 0%, rgba(210,182,148,1) 50%, rgba(0,0,0,0) 100%);
background: -o-linear-gradient(0deg, rgba(0,0,0,0) 0%, rgba(210,182,148,1) 50%, rgba(0,0,0,0) 100%);
background: -ms-linear-gradient(0deg, rgba(0,0,0,0) 0%, rgba(210,182,148,1) 50%, rgba(0,0,0,0) 100%);
background: linear-gradient(90deg, rgba(0,0,0,0) 0%, rgba(210,182,148,1) 50%, rgba(0,0,0,0) 100%);
filter: progid:DXImageTransform.Microsoft.gradient( startColorstr='#000000', endColorstr='#000000',GradientType=1 );
}
.ip-ul1 li:last-child:before {
display: none;
}
.ip-ulp1,
.ip-ulp2{
display: table-cell;
width: 50%;
vertical-align: middle;
padding: 0 3px;
}
.ip-ulp2 span{
display: block;
font-size: 15px;
line-height: 1.47;
text-align: center;
color: #ffe5db;
}
.ip-ulp2 span:nth-child(2){
padding-bottom: 10px;
}
.mi-btn1,
.ip-tit2m,
.ip-ul1{
display: none;
}
@media (max-width: 1200px) {
.mi-mns li {
margin-left: 40px;
}
.ip-btn1,
.ip-tit1{
padding: 0;
}
.ip-tit2{
font-size: 38px;
}
}
@media (max-width: 992px) {
.mi-btn1,
.ip-tit2m,
.ip-ul1{
display: block;
}
.ip-tit2,
.ip-img1,
.ip-btn1{
display: none;
}
.ip-tit1{
font-size: 17px;
padding: 30px 0 10px 0;
}
}
@media (max-width: 450px) {
.ip-ulp2 span{
font-size: 12px;
}
.ip-btna {
font-size: 16px;
height: 35px;
line-height: 35px;
padding: 0 20px;
}
.ip-tit2m{
font-size: 30px;
}
.ip-tit1{
font-size: 13px;
}
}
</style>
<!--15112018-->
<style>
@media (max-width: 1200px) {
.ip-btna {
font-size: 15px;
padding: 0 25px;
}
}
</style>
<div class="xs-main">
<div class="ip-s1">
<div class="container">
<div class="col-md-4">
<p class="ip-s1i1"><img src="images/2610/phone.png" alt=""></p>
</div>
<div class="col-md-8">
<p class="ip-tit1">iPhone XS <span>|</span> iPhone XS Max <span>|</span> iPhone XR</p>
<p class="ip-tit2"><span>Lớn</span> tối đa - <span>Vàng</span> phong cách</p>
<p class="ip-tit2m">Lớn tối đa</p>
<p class="ip-tit2m">Vàng phong cách</p>
<p class="ip-img1"><img src="images/1511/s1.png" alt=""></p>
<p class="ip-btn1">
<a href="" title="" class="ip-btna">Mua ngay</a>
<a href="" title="" class="ip-btna">Mua ngay</a>
<a href="" title="" class="ip-btna">Mua ngay</a>
<a href="" title="" class="ip-btna">Mua ngay</a>
</p>
<ul class="ip-ul1">
<li>
<p class="ip-ulp1"><img src="images/1511/1.png" alt=""></p>
<p class="ip-ulp2"><span>Trả thẳng</span><span>giảm đến 2 triệu</span> <a href="" title="" class="ip-btna">Mua ngay</a></p>
</li>
<li>
<p class="ip-ulp1"><img src="images/1511/2.png" alt=""></p>
<p class="ip-ulp2"><span></span><span>Trả góp 0%</span><a href="" title="" class="ip-btna">Mua ngay</a></p>
</li>
<li>
<p class="ip-ulp1"><img src="images/1511/3.png" alt=""></p>
<p class="ip-ulp2"><span>Hoàn 3 triệu + Trả góp 0%</span><span>Chủ thẻ tín dụng Citibank</span><a href="" title="" class="ip-btna">Mua ngay</a></p>
</li>
<li>
<p class="ip-ulp1"><img src="images/1511/4.png" alt=""></p>
<p class="ip-ulp2"><span>Thu cũ - Đổi mới</span><span> Tiết kiệm đến 12 triệu</span> <a href="" title="" class="ip-btna">Mua ngay</a></p>
</li>
</ul>
</div>
</div>
</div>
<div class="mi-menu">
<div class="container">
<p class="mi-lg"><img src="images/1310/lg-mn.png" alt=""></p>
<ul class="mi-mns clearfix">
<li><a toscroll="" href="javascript: void(0)" title="">Phương thức mua hàng</a></li>
<li><a toscroll=".xs-s2" href="javascript: void(0)" title="">Thông tin sản phẩm</a></li>
<li><a toscroll="#thele" href="javascript: void(0)" title="">Thể lệ</a></li>
<li><a toscroll="#binhluan" href="javascript: void(0)" title="">Bình luận</a></li>
</ul>
<a class="mi-btn1" href="#MuaNgay" data-toggle="modal" title="" toscroll="#hinhanh">Đăng ký</a>
</div>
</div>
<style>
.xs-mdclose{
position: absolute;
top: 10px;
right: 10px;
display: inline-block;
-webkit-transform: rotate(45deg);
-moz-transform: rotate(45deg);
-ms-transform: rotate(45deg);
-o-transform: rotate(45deg);
transform: rotate(45deg);
}
.xs-mdclose span{
display: inline-block;
width: 30px;
height: 30px;
position: relative;
cursor: pointer;
}
.xs-mdclose span:before{
content: "";
display: inline-block;
width: 30px;
height: 2px;
background: #000000;
position: absolute;
top: 50%;
left: 0;
margin-top: -1px;
}
.xs-mdclose span:after{
content: "";
display: inline-block;
height: 30px;
width: 2px;
background: #000000;
position: absolute;
left: 50%;
top: 0;
margin-left: -1px;
}
.xsvd-content{
width: 100%;
height: 100%;
position: relative;
padding: 40px 0;
display: -webkit-box;
display: -ms-flexbox;
display: flex;
-webkit-box-pack: center;
-ms-flex-pack: center;
justify-content: center;
}
#xsvdmd{
background: #fff;
}
.xs-playsbox{
max-width: 1280px;
width: auto;
min-width: 320px;
display: -webkit-box;
display: -ms-flexbox;
display: flex;
position: relative;
}
.xs-playmain{
width: auto;
height: 100vh;
max-height: 720px;
overflow: hidden;
position: relative;
-ms-flex-item-align: center;
align-self: center;
}
.ac-video-media-controller{
width: auto;
min-width: 320px;
max-height: 100vh;
max-width: 100vw;
display: block;
outline: none;
}
.xs-playmain>* {
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
</style>
<div class="modal fade" id="xsvdmd" tabindex="-1" role="dialog">
<div class="xsvd-content">
<p class="xs-mdclose" data-dismiss="modal"><span></span></p>
<div class="xs-playsbox">
<div class="xs-playmain">
<video class="ac-video-media-controller" controls>
<source src="https://www.apple.com/105/media/us/iphone-xs/2018/674b340a-40f1-4156-bbea-00f386459d3c/films/design/iphone-xs-design-tpl-cc-us-2018_1280x720h.mp4" type="video/mp4" media="all and (max-width:1200px)">
<source src="https://www.apple.com/105/media/us/iphone-xs/2018/674b340a-40f1-4156-bbea-00f386459d3c/films/design/iphone-xs-design-tpl-cc-us-2018_1280x720h.mp4" type="video/mp4">
</video>
</div>
</div>
</div>
</div>
<div class="xs-s3">
<div class="xs-s3play">
<p class="xs-s3b">iPhone thế hệ mới.</p>
<a class="xs-s3t" href="#xsvdmd" title="" data-toggle="modal">Xem video <span class="glyphicon glyphicon-play-circle"></span></a>
</div>
<p class="xs-s3i"><img src="images/1310/s2.png" alt=""></p>
</div>
<!--26/10/2018-->
<style>
.ip-tit{
font-size: 56px;
}
.ip-sub{
font-size: 27px;
}
.xs-main{
background: #000000;
}
.mi-menu{
background: #111111;
}
.ip-tit22{
font-size: 28px;
color: #ffffff;
padding-top: 40px;
}
.ip-tit3{
font-size: 56px;
color: #ffffff;
font-weight: bold;
padding-bottom: 20px;
}
.ip-sub2{
font-size: 27px;
line-height: 1.41;
font-weight: bold;
color: #ffffff;
}
.ip-s3tit2{
font-size: 36px;
color: #ffffff;
font-weight: bold;
}
.ip-st .ip-s3sub{
max-width: 460px;
}
.ip-tskt{
text-align: center;
padding: 60px 0 80px 0;
}
.ip-tsul{
display: flex;
justify-content: space-evenly;
max-width: 488px;
margin: 0 auto;
border: 1px solid #d6d6d6;
-webkit-border-radius: 4px;
-moz-border-radius: 4px;
border-radius: 4px;
}
.ip-tsul li{
display: inline-block;
height: 45px;
line-height: 45px;
width: 33.34%;
border-right: 1px solid #d6d6d6;
}
.ip-tsul li:last-child
{
border: none;
}
.ip-tsul li.active{
background: linear-gradient(to top, #B18667 0%, #FADFCC 100%);
background: -moz-linear-gradient(to top, #B18667 0%, #FADFCC 100%);
background: -o-linear-gradient(to top, #B18667 0%, #FADFCC 100%);
background: -ms-linear-gradient(to top, #B18667 0%, #FADFCC 100%);
background: -webkit-linear-gradient(bottom, #B18667 0%, #FADFCC 100%);
}
.ip-tsul li.active a{
color: #000000;
}
.ip-tsul li a{
font-size: 18px;
line-height: 1.43;
color: #f2f2f2;
}
.ip-color{
margin: 20px 0 15px 0;
}
.ip-color li{
display: inline-block;
vertical-align: middle;
padding: 0 10px;
}
.ip-color li span{
display: inline-block;
cursor: pointer;
width: 20px;
height: 20px;
border: solid 1px #979797;
-webkit-border-radius: 100%;
-moz-border-radius: 100%;
border-radius: 100%;
background-color: #d8d8d8;
}
.ip-color li:nth-child(2) span{
background-color: #c9a488;
}
.ip-color li:nth-child(3) span{
background-color: #373737;
}
.ip-cl{
font-size: 15px;
color: #ffe5db;
}
.ip-gia span:last-child{
font-size: 20px;
font-weight: bold;
color: #ffe5db;
padding-left: 10px;
}
.ip-btnts a{
display: inline-block;
font-size: 24px;
line-height: 50px;
height: 50px;
color: #000000;
padding: 0 40px;
margin-top: 15px;
-webkit-border-radius: 6px;
-moz-border-radius: 6px;
border-radius: 6px;
background: linear-gradient(to top, #B18667 0%, #FADFCC 100%);
background: -moz-linear-gradient(to top, #B18667 0%, #FADFCC 100%);
background: -o-linear-gradient(to top, #B18667 0%, #FADFCC 100%);
background: -ms-linear-gradient(to top, #B18667 0%, #FADFCC 100%);
background: -webkit-linear-gradient(bottom, #B18667 0%, #FADFCC 100%);
}
.ip-tabcl{
padding-top: 55px;
}
.nk6-s6hd{
font-size: 28px;
font-weight: bold;
color: #ffe5db;
margin-bottom: 15px;
text-align: left;
}
.nk6-s6ts{
text-align: left;
}
.nk6-s6ts li{
display: table;
width: 100%;
padding: 10px 0;
font-size: 15px;
}
.nk6-s6ts li:last-child{
border-bottom: none;
}
.nk6-s6ts li label{
display: table-cell;
vertical-align: top;
width: 180px;
font-weight: bold;
color: #ffffff;
}
.nk6-s6ts li span{
display: table-cell;
vertical-align: top;
color: #ffe5db;
}
.ip-clxt a{
display: inline-block;
height: 35px;
line-height: 35px;
font-size: 17px;
text-align: center;
color: #ffe5db;
padding: 0 20px;
margin-top: 15px;
-webkit-border-radius: 4px;
-moz-border-radius: 4px;
border-radius: 4px;
border: solid 1px #4a4a4a;
background-color: #111111;
}
@media (max-width: 992px) {
.ip-tskt{
padding: 50px 20px;
}
.nk6-s6hd{
padding-top: 60px;
}
.ip-tsul li{
height: 36px;
line-height: 36px;
}
.ip-tit,
.ip-tit3{
font-size: 36px;
}
.ip-tit1,
.ip-tit22{
font-size: 17px;
padding: 0 0 20px 0;
}
.ip-sub{
font-size: 22px;
}
.ip-s3{
padding: 50px 20px;
}
.ip-s3tit2{
font-size: 26px;
}
.ip-st{
padding-top: 20px;
}
}
@media (max-width: 550px) {
.ip-tsul li a{
font-size: 12px;
}
}
@media (max-width: 450px) {
.nk6-s6ts li label{
width: 120px;
}
.ip-tit3{
font-size: 28px;
}
.ip-sub2,
.ip-sub{
font-size: 18px;
}
}
</style>
<div class="ip-s3">
<div class="ip-sw">
<p class="ip-tit1">iPhone XS <span>|</span> iPhone XS Max</p>
<p class="ip-tit">Thế giới màn hình lớn chào đón bạn</p>
<p class="ip-sub">Xin giới thiệu chiếc iPhone màn hình lớn nhất từ trước đến nay khi bạn sẽ có hai lựa chọn kích thước màn hình khác nhau, cùng ở chuẩn độ phân giải Super Retina siêu sắc nét. Face ID cực nhanh, con chip mạnh mẽ và thông minh nhất thế giới smartphone, hệ thống camera kép đột phá, iPhone Xs tập hợp những điều bạn yêu thích nhất trên một chiếc iPhone.</p>
<div class="ip-s3tab">
<div class="row">
<div class="col-md-6">
<p class="ip-s3tit">Màn hình</p>
<p class="ip-s3sub">Lớn nhất 6.5 inch dù kích thướctổng thể máy vật lý không thay đổi</p>
<p class="ip-s3i"><img src="images/2610/s311.png" alt=""></p>
</div>
<div class="col-md-6">
<p class="ip-s3tit">Mầu sắc</p>
<p class="ip-s3sub">Vàng mới phong cách, khung viền thép sáng bóng, lưng kính cường lực</p>
<p class="ip-s3i"><img src="images/1310/s32.png" alt=""></p>
</div>
<div class="col-md-6">
<p class="ip-s3tit">Camera</p>
<p class="ip-s3sub">iPhone chính là “máy ảnh” phổ biến nhất thế giới trong những năm qua</p>
<p class="ip-s3i"><img src="images/1310/s33.png" alt=""></p>
</div>
<div class="col-md-6">
<p class="ip-s3tit">Cấu hình</p>
<p class="ip-s3sub">A12 Bionic là chip mạnh mẽ nhất trong thế giới điện thoại thông minh</p>
<p class="ip-s3i"><img src="images/1310/s34.png" alt=""></p>
</div>
</div>
</div>
</div>
</div>
<div class="ip-s3 ip-st">
<div class="ip-sw">
<p class="ip-tit22">iPhone XR</p>
<p class="ip-tit3">Xuất sắc. Trên mọi phương diện</p>
<p class="ip-sub2">Thiết kế màn hình mới với Liquid Retina 6.1” - loại màn hình LCD tiên tiến nhất. Cùng Face ID cực nhanh, con chip A12 Bionic mạnh mẽ và thông minh nhất thế giới Smartphone, hệ thống camera 12MP đột phá, iPhone XR đẹp dưới mọi góc nhìn</p>
<div class="ip-s3tab">
<div class="row">
<div class="col-md-6">
<p class="ip-s3tit2">Màn hình</p>
<p class="ip-s3sub">Thiết kế toàn màn hình với Liquid Retina 6.1” là màn hình LCD tiên tiến nhất thế giới</p>
<p class="ip-s3i"><img src="images/2610/s31.png" alt=""></p>
</div>
<div class="col-md-6">
<p class="ip-s3tit2">Mầu sắc</p>
<p class="ip-s3sub">Có 6 mầu với mặt lưng kính tạo nên sự bóng bẩy, trẻ trung, năng động</p>
<p class="ip-s3i"><img src="images/2610/s32.png" alt=""></p>
</div>
<div class="col-md-6">
<p class="ip-s3tit2">Camera</p>
<p class="ip-s3sub">12 MP, f/1.8 chụp được xoá phông và điều chỉnh độ mờ hậu cảnh sau chụp</p>
<p class="ip-s3i"><img src="images/2610/s33.png" alt=""></p>
</div>
<div class="col-md-6">
<p class="ip-s3tit2">Cấu hình</p>
<p class="ip-s3sub">Memoji, Face ID nhanh hơn, Chip A12 Bionic mạnh hơn như trên iPhone Xs</p>
<p class="ip-s3i"><img src="images/2610/s34.png" alt=""></p>
</div>
</div>
</div>
</div>
</div>
<div class="ip-tskt">
<div class="ip-sw">
<ul class="ip-tsul">
<li class="active"><a data-toggle="tab" href="#iphonexsmax">iPhone XS Max</a></li>
<li><a data-toggle="tab" href="#iphonexs">iPhone XS</a></li>
<li><a data-toggle="tab" href="#iphonexr">iPhone XR</a></li>
</ul>
<div class="tab-content ip-tabcl">
<div id="iphonexsmax" class="tab-pane active">
<div class="row">
<div class="col-md-6">
<p><img src="images/2610/choose.png" alt=""></p>
<ul class="ip-color">
<lable class="ip-cl">Màu sắc:</lable>
<li><span></span></li>
<li><span></span></li>
<li><span></span></li>
</ul>
<p class="ip-gia"><span class="ip-cl">Giá từ:</span> <span>33.990.000₫</span></p>
<p class="ip-btnts"><a href="" title="">Đặt hàng ngay</a></p>
</div>
<div class="col-md-6">
<h2 class="nk6-s6hd">Thông số kỹ thuật</h2>
<ul class="nk6-s6ts">
<li><label>Màn hình:</label> <span>5.5 inch Full HD (1080 x 1920 pixels)</span></li>
<li><label>Thiết kế:</label> <span>Thiết kế Khung kim loại + mặt kính cường lực</span></li>
<li><label>Camera sau:</label> <span>16.0 MP (f2.0)</span></li>
<li><label>Camera trước:</label> <span>8.0 MP (f2.0)</span></li>
<li><label>Tính năng camera:</label> <span>Camera sau: Tự động lấy nét, Chạm lấy nét, Nhận diện khuôn mặt, HDR, Panorama
Camera trước: Chế độ làm đẹp, Camera góc rộng, Nhận diện khuôn mặt
</span></li>
<li><label>CPU:</label> <span>Qualcomm Snapdragon 430, 8 Nhân</span></li>
<li><label>RAM:</label> <span>3 GB</span></li>
<li><label>Bộ nhớ lưu trữ:</label> <span>32 GB</span></li>
<li><label>Hỗ trợ thẻ nhớ:</label> <span>MicroSD lên tới 128 GB</span></li>
<li><label>Thẻ sim:</label> <span>2 Sim (Nano SIM)</span></li>
<li><label>Dung lượng pin:</label> <span>3000mAh</span></li>
<li><label>Trọng lượng:</label> <span>169 g</span></li>
<li><label>Kết nối mạng:</label> <span>LTE</span></li>
<li><label>Cảm biến:</label> <span>Mở khoá vân tay</span></li>
</ul>
<p class="ip-clxt"><a href="" title="">Xem đầy đủ thông số</a></p>
</div>
</div>
</div>
<div id="iphonexs" class="tab-pane">
<div class="row">
<div class="col-md-6">
<p><img src="images/2610/choose.png" alt=""></p>
<ul class="ip-color">
<lable class="ip-cl">Màu sắc:</lable>
<li><span></span></li>
<li><span></span></li>
<li><span></span></li>
</ul>
<p class="ip-gia"><span class="ip-cl">Giá từ:</span> <span>33.990.000₫</span></p>
<p class="ip-btnts"><a href="" title="">Đặt hàng ngay</a></p>
</div>
<div class="col-md-6">
<h2 class="nk6-s6hd">Thông số kỹ thuật</h2>
<ul class="nk6-s6ts">
<li><label>Màn hình:</label> <span>5.5 inch Full HD (1080 x 1920 pixels)</span></li>
<li><label>Thiết kế:</label> <span>Thiết kế Khung kim loại + mặt kính cường lực</span></li>
<li><label>Camera sau:</label> <span>16.0 MP (f2.0)</span></li>
<li><label>Camera trước:</label> <span>8.0 MP (f2.0)</span></li>
<li><label>Tính năng camera:</label> <span>Camera sau: Tự động lấy nét, Chạm lấy nét, Nhận diện khuôn mặt, HDR, Panorama
Camera trước: Chế độ làm đẹp, Camera góc rộng, Nhận diện khuôn mặt
</span></li>
<li><label>CPU:</label> <span>Qualcomm Snapdragon 430, 8 Nhân</span></li>
<li><label>RAM:</label> <span>3 GB</span></li>
<li><label>Bộ nhớ lưu trữ:</label> <span>32 GB</span></li>
<li><label>Hỗ trợ thẻ nhớ:</label> <span>MicroSD lên tới 128 GB</span></li>
<li><label>Thẻ sim:</label> <span>2 Sim (Nano SIM)</span></li>
<li><label>Dung lượng pin:</label> <span>3000mAh</span></li>
<li><label>Trọng lượng:</label> <span>169 g</span></li>
<li><label>Kết nối mạng:</label> <span>LTE</span></li>
<li><label>Cảm biến:</label> <span>Mở khoá vân tay</span></li>
</ul>
<p class="ip-clxt"><a href="" title="">Xem đầy đủ thông số</a></p>
</div>
</div>
</div>
<div id="iphonexr" class="tab-pane">
<div class="row">
<div class="col-md-6">
<p><img src="images/2610/choose.png" alt=""></p>
<ul class="ip-color">
<lable class="ip-cl">Màu sắc:</lable>
<li><span></span></li>
<li><span></span></li>
<li><span></span></li>
</ul>
<p class="ip-gia"><span class="ip-cl">Giá từ:</span> <span>33.990.000₫</span></p>
<p class="ip-btnts"><a href="" title="">Đặt hàng ngay</a></p>
</div>
<div class="col-md-6">
<h2 class="nk6-s6hd">Thông số kỹ thuật</h2>
<ul class="nk6-s6ts">
<li><label>Màn hình:</label> <span>5.5 inch Full HD (1080 x 1920 pixels)</span></li>
<li><label>Thiết kế:</label> <span>Thiết kế Khung kim loại + mặt kính cường lực</span></li>
<li><label>Camera sau:</label> <span>16.0 MP (f2.0)</span></li>
<li><label>Camera trước:</label> <span>8.0 MP (f2.0)</span></li>
<li><label>Tính năng camera:</label> <span>Camera sau: Tự động lấy nét, Chạm lấy nét, Nhận diện khuôn mặt, HDR, Panorama
Camera trước: Chế độ làm đẹp, Camera góc rộng, Nhận diện khuôn mặt
</span></li>
<li><label>CPU:</label> <span>Qualcomm Snapdragon 430, 8 Nhân</span></li>
<li><label>RAM:</label> <span>3 GB</span></li>
<li><label>Bộ nhớ lưu trữ:</label> <span>32 GB</span></li>
<li><label>Hỗ trợ thẻ nhớ:</label> <span>MicroSD lên tới 128 GB</span></li>
<li><label>Thẻ sim:</label> <span>2 Sim (Nano SIM)</span></li>
<li><label>Dung lượng pin:</label> <span>3000mAh</span></li>
<li><label>Trọng lượng:</label> <span>169 g</span></li>
<li><label>Kết nối mạng:</label> <span>LTE</span></li>
<li><label>Cảm biến:</label> <span>Mở khoá vân tay</span></li>
</ul>
<p class="ip-clxt"><a href="" title="">Xem đầy đủ thông số</a></p>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="ip-s4">
<div class="ip-sw">
<div class="ip-s4w">
<p class="ip-s4i"><img src="images/1310/s41.png" alt=""></p>
<div class="ip-s4c">
<p><img src="images/1310/s42.png" alt=""></p>
<p class="ip-btn"><a href="ip-btn">Đặt ngay</a></p>
<p class="ip-s1c">Giá từ 11.999.000đ</p>
</div>
</div>
</div>
</div>
<div class="ss-tlds" id="thele">
<div class="container">
<div class="row">
<div class="col-md-6 col-sm-12">
<h3 class="p1-s4tit">Thể lệ chương trình</h3>
<div class="p1-s4ttle mCustomScrollbar">
<p><strong>Thời gian:</strong>Áp dụng từ 05/04/2016</p>
<p><strong>Ưu đãi:</strong> khách hàng mua Galaxy S7, S7 edge nhận được tới <strong>9 ưu đãi khủng</strong>:</p>
<ul>
<li><strong>1:</strong> Trả góp Home Credit 0% lãi suất, trả trước
40%,
kỳ hạn 6 tháng </li>
<li><strong>2:</strong> Tặng Sạc dự phòng nhanh Samsung 5200mAh
(không
áp dụng trả góp 0%)</li>
<li><strong>3:</strong> Sim Đại Phát 0888xxxxx8 (không áp dụng trả
góp
0%)</li>
<li><strong>4:</strong> 3 tháng cước 3G trị giá 210.000đ (không áp
dụng
trả góp 0%)</li>
<li><strong>5:</strong> Phiếu mua hàng 220.000đ áp dụng mua Sạc dự
phòng
6000mAh hoặc 10000mAh</li>
<li><strong>6:</strong> Gói chăm sóc đặc quyền: 1 đổi 1, hỗ trợ kỹ
thuật
tận nhà, Hotline VIP</li>
<li><strong>7:</strong> Gói ưu đãi đặc quyền: phòng chờ máy bay hạng
thương gia, cafe/xem phim miễn phí rạp CGV, gói game-pack trị giá
200USD...></li>
<li><strong>8:</strong> Cơ hội trúng thưởng 27 Galaxy S7</li>
<li><strong>9:</strong> Cơ hội trúng thưởng 300 Tivi Samsung 32”</li>
</ul>
<p><strong>Thời gian mở bán:</strong>Từ 18/03/2016</p>
<p><strong>Ưu đãi:</strong> khách hàng mua Galaxy S7, S7 edge chọn 1 trong 3 gói khuyến mãi sau:</p>
<ul>
<li><strong>KM1:</strong> Trả góp Home Credit 0% lãi suất, trả trước 40%, kỳ hạn 6 tháng</li>
<li><strong>KM2:</strong> Tặng Sạc dự phòng nhanh Samsung 5200mAh + Sim Đại Phát 088</li>
<li><strong>KM3:</strong> Trả góp 0% bằng thẻ tín dụng + Sạc dự phòng nhanh Samsung 5200mAh + Sim Đại Phát 088 (áp dụng từ 14h 22/03)</li>
</ul>
<p><strong>Ngoài 1 trong 3 gói khuyến mãi trên, khách hàng nhận thêm:</strong></p>
<ul>
<li><strong>Gói ưu đãi đặc quyền:</strong> phòng chờ máy bay hạng thương gia, cafe/xem phim miễn phí rạp CGV, gói game trị giá 200USD...
<li><strong>Gói chăm sóc đặc quyền:</strong> 1 đổi 1, hỗ trợ kỹ thuật tận nhà, Hotline VIP</li>
<li><strong>Cơ hội trúng thưởng 27 Galaxy S7</strong> dành cho kháchhàng mua hàng từ 01/03 - 30/04 (không áp dụng cho khách hàng đã trúng thưởng đợt 1).</li>
<li><strong>Khuyến mãi phụ kiện giảm tới 30%</strong> khi mua kèm</li>
</ul>
<p><strong>Lưu ý:</strong>Không áp dụng đối với đơn hàng đặt cọc từ 01/03 đến 17/03.</p>
<p><strong>Thời gian đặt cọc: </strong>01/03/2016 - 22h00 17/03/2016</p>
<p><strong>Ưu đãi: </strong></p>
<p><strong>- Từ 01/03 đến 12h00 03/03:</strong> khách hàng may mắn có cơ hội nhận được</p>
</div>
</div>
<div class="col-md-6 col-sm-12">
<h3 class="p1-s4tit">Có <strong>132</strong> người chơi tham gia</h3>
<ul class="p1-s4ulsh clearfix">
<li>
<div class="p1-s4search">
<input type="text" class="form-control" placeholder="Nhập số điện thoại để tìm kiếm">
</div>
</li>
<li>
<input type="checkbox" class="p1-s4rad" id="p1s4rad5">
<label for="p1s4rad5" class="p1-s4lb">Chỉ hiển thị người trúng giải</label>
</li>
</ul>
<div class="p1-s4table mCustomScrollbar">
<table class="table table-hover table-striped">
<thead>
<tr>
<td>STT</td>
<td>Họ tên</td>
<td>Điện thoại</td>
<td>Trạng thái</td>
</tr>
</thead>
<tbody>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
<tr>
<td>123</td>
<td>Nguyễn Văn Anh</td>
<td>0986222xxx</td>
<td>Đã cọc</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
<div id="binhluan">
</div>
</div>
<footer class="of-footer">
<div class="container">
<div class="of-footer-link">
<a target="_blank" href="http://fptshop.com.vn/huong-dan/huong-dan-mua-hang" title="Hướng dẫn mua hàng">Hướng dẫn mua hàng</a> |
<a target="_blank" href="http://fptshop.com.vn/ho-tro/chinh-sach-tra-gop" title="Trả góp">Trả góp</a> |
<a target="_blank" href="http://fptshop.com.vn/ho-tro/chinh-sach-bao-hanh" title="Chính sách bảo hành">Chính sách bảo hành</a>
</div>
<p>Công Ty Cổ Phần Bán Lẻ Kỹ Thuật Số FPT - 261-263 Khánh Hội, P5, Q4, TP. Hồ Chí Minh.</p>
<p>Điện thoại: 028.73023456. Fax: 028.39435773. Email: <EMAIL></p>
</div>
</footer>
</body>
</html>
<file_sep>(function($){
var slickNews = {
init: function () {
slickNews.events();
},
events: function () {
$('.of9-s2wrap').countdown('2018/08/15 00:00', function(event) {
$(this).html(event.strftime('<span class="glyphicon glyphicon-time of9-s2icon"></span> <span class="of9-s2tit">Oppo F9 sẽ ra mắt sau</span> <i></i> <span class="of9-s2time of9-s2tm1">%D</span> <span class="of9-s2tit">ngày</span> <span class="of9-s2time of9-s2tm2">%H:%M:%S</span>'));
});
}
};
$(document).ready(function (){
$('.of-iconmntop').click(function(){
$('.of-menutop').toggleClass('of-mnshow');
});
$('.of-search').click(function(){
$('.of-searchbox').show(200);
});
$('.of-closesearch').click(function(){
$('.of-searchbox').hide(200);
});
//landing
if ($(".of9-s2wrap").length > 0) {
slickNews.init();
}
$(document).on('click','[toscroll]',function(e){
e.preventDefault();
var link = $(this).attr('toscroll');
if($(link).length > 0){
var posi = $(link).offset().top - 50;
$('body,html').animate({scrollTop:posi},1000);
}
});
$(window).bind('scroll', function () {
if ($(window).scrollTop() > 70) {
$('.of9-menu').addClass('of9-mnfix');
} else {
$('.of9-menu').removeClass('of9-mnfix');
}
});
var swiper = new Swiper('.swiper-s7', {
slidesPerView: 4,
spaceBetween: 20,
pagination: {
el: '.swiper-pagination',
clickable: true,
},
breakpoints: {
768: {
slidesPerView: 3,
spaceBetween: 10,
},
640: {
slidesPerView: 2,
spaceBetween: 5,
},
374: {
slidesPerView: 1,
spaceBetween: 0,
}
}
});
$(document).on('click','.of9-s4ldo',function () {
$('.of9-s4mau').hide();
$('.of9-s4do').show();
});
$(document).on('click','.of9-s4lxanh',function () {
$('.of9-s4mau').hide();
$('.of9-s4xanh').show();
});
/*09082018*/
$(document).on('click','.of9-vd2 span',function (ev) {
$(".of9-vdplay").slideDown();
$(".of9-video").slideUp();
$(".of9-vdplay iframe")[0].src += "&autoplay=1";
ev.preventDefault();
});
// 10082018
var swiper12 = new Swiper('.swiper-s91', {
effect: 'coverflow',
grabCursor: true,
loop: true,
loopFillGroupWithBlank: true,
centeredSlides: true,
slidesPerView: 'auto',
coverflowEffect: {
rotate: 0,
stretch: 0,
depth: 100,
modifier: 1,
slideShadows : true,
},
navigation: {
nextEl: '.swiper-s91 .swiper-button-next',
prevEl: '.swiper-s91 .swiper-button-prev',
}
});
var swiper13 = new Swiper('.swiper-s92', {
effect: 'coverflow',
grabCursor: true,
loop: true,
loopFillGroupWithBlank: true,
centeredSlides: true,
slidesPerView: 'auto',
coverflowEffect: {
rotate: 0,
stretch: 0,
depth: 100,
modifier: 1,
slideShadows : true,
},
navigation: {
nextEl: '.swiper-s92 .swiper-button-next',
prevEl: '.swiper-s92 .swiper-button-prev',
}
});
$(document).on('click','#of9-s9btnd',function () {
$('.of9-s9ul li').removeClass("active");
$(this).parent().addClass('active');
$('.of9-s9slide').hide();
$('.of9-s9do').show();
swiper12.update();
});
$(document).on('click','#of9-s9btnx',function () {
$('.of9-s9ul li').removeClass("active");
$(this).parent().addClass('active');
$('.of9-s9slide').hide();
$('.of9-s9xanh').show();
swiper13.update();
});
});
})(window.jQuery); | fff4459e00ccbb295b9ddd75227738450796ce6d | [
"JavaScript",
"HTML"
] | 7 | JavaScript | Nhungpt6/Demo | f230c2f78131280ad2aef41e1eff0020cacaab97 | b510213ae4d1761bb6540966ca5efef687c642f6 |
refs/heads/master | <repo_name>jameswilding/iphoneification<file_sep>/init.rb
require 'iphoneification'
# iPhones really get plain old HTML, but we need a way to distinguish them from regular browsers.
Mime::Type.register_alias "text/html", :iphone
if defined?(ActionController::Base)
ActionController::Base.send(:extend, IPhoneification::ClassMethods)
ActionController::Base.send(:include, IPhoneification::InstanceMethods)
end<file_sep>/lib/iphoneification.rb
module IPhoneification
# Class methods are added to ActionController::Base so you can do:
#
# class ApplicationController < ActionController::Base
# responds_to_iphone
# ...
# end
#
# Use +responds_to_iphone!+ (with the bang) instead to make your app act
# like an Apple fanboy, always serving iPhone views even if your visitors
# are using some other browser.
module ClassMethods
# #responds_to_iphone sets up the before-filter which checks for iphone requests
# and adjusts the request format accordingly.
#
# You can then use +respond_to+ in your controllers to serve
# iPhone-optimised views:
#
# def index
# @posts = Post.all
#
# respond_to do |format|
# format.html # renders posts/index.html.erb
# format.iphone { some_special_code_for_iphone_requests } # renders posts/index.iphone.erb
# end
# end
#
# Use the extension '.iphone.erb' for your iPhone views and templates.
#
# ==== Options
# Pass in any options appropriate for +before_filter+.
def responds_to_iphone(options = {})
before_filter :adjust_format_for_iphone_requests, options
end
# Makes a controller act like _every_ request is from an iPhone.
#
# ==== Options
# Pass in any options appropriate for +before_filter+.
def responds_to_iphone!(options = {})
before_filter :ensure_format_is_iphone, options
end
# Skips the iphoneification before_filter set by #responds_to_iphone or #responds_to_iphone!.
#
# ==== Options
# Pass in any options appropriate for +before_filter+.
def skip_iphone_response(options = {})
if self.filter_chain.any? { |f| f.method == :ensure_format_is_iphone }
skip_before_filter :ensure_format_is_iphone, options
else
skip_before_filter :adjust_format_for_iphone_requests, options
end
end
end
# Just some utility methods.
module InstanceMethods
private
# Sets request.format to :iphone if request is from an iPhone.
def adjust_format_for_iphone_requests
set_format_to_iphone if iphone_request?
end
# Just sets format to :iphone, whatever.
def ensure_format_is_iphone
set_format_to_iphone # always
end
# Rails uses request.format in +respond_to+ blocks, and other places:
# this is where the magic happens.
def set_format_to_iphone
request.format = :iphone
end
# This method looks at the browser's user-agent string to determine
# whether or not the current request is from an iPhone (or iPod Touch).
def iphone_request?
(agent = request.env["HTTP_USER_AGENT"]) && (agent[/(Mobile\/.+Safari)/] || agent[/iPhone/])
end
end
end<file_sep>/README.md
# iphoneification
A Rails plugin which lets your app serve custom content to iPhone and iPod Touch users.
## Usage
script/plugin install git://github.com/jameswilding/iphoneification.git
class ApplicationController
responds_to_iphone
end
Name your views and layouts {name}.iphone.erb. Skip iphonification with <code>skip_iphone_response</code>. The <code>responds_to_iphone and skip_iphone_response</code> methods takes the same options as <code>before_filter</code> and <code>skip_before_filter</code>, respectively: see [api.rubyonrails.org][api] for more details.
## More
Original blog post at [http://jameswilding.net/2009/08/10/iphone-on-rails/][blog].
## Credit
Based on [an article from slashdotdash.net][slash].
## Copyright
Copyright (c) 2009 <NAME>, released under the MIT license.
[api]: http://api.rubyonrails.org/
[blog]: http://jameswilding.net/2009/08/10/iphone-on-rails/
[slash]: http://www.slashdotdash.net/2007/12/04/iphone-on-rails-creating-an-iphone-optimised-version-of-your-rails-site-using-iui-and-rails-2/<file_sep>/test/iphoneification_test.rb
require 'test_helper'
# No tests yet.
class IphoneificationTest < ActiveSupport::TestCase
# Replace this with your real tests.
test "the truth" do
assert true
end
end
| cbbfee9686cdc0e11dc1dde04817ad97009609b0 | [
"Markdown",
"Ruby"
] | 4 | Ruby | jameswilding/iphoneification | 747df8a1bc0fba9ad35957b63cb2b807d8b1f5f0 | b3a610759d713f29a3d9bdc272d7ff71ba7180e3 |
refs/heads/master | <repo_name>parallaxisjones/rdm-search-docker-compose<file_sep>/mappings/README.md
# ElasticSearch Mapping files for RDM objects
- `mapping.json`: mapping file for the `rdm` index of elastic
## create index
```bash
$ curl -XPUT -d @mapping.json http://<elastic-server>:9200/rdm
```
<file_sep>/scripts/elasticsearch_post_startup.sh
#!/bin/sh
ELASTIC_ENDPOINT="http://elasticsearch:9200"
elasticsearch_post_startup() {
echo Put index templates
curl -s -w "\n" -XPUT "${ELASTIC_ENDPOINT}/_template/filebeat" -d @/config/indices/templates/filebeat.template.json
echo Put index mappings
# curl -s -w "\n" -XDELETE "${ELASTIC_ENDPOINT}/rdm"
if !(curl -s -f "${ELASTIC_ENDPOINT}/rdm" &> /dev/null); then
echo Create index rdm
curl -s -w "\n" -XPUT "${ELASTIC_ENDPOINT}/rdm" -d @/config/indices/rdm/settings.json
fi
echo Close index rdm
curl -s -w "\n" -XPOST "${ELASTIC_ENDPOINT}/rdm/_close"
echo Updating analyzers for rdm
curl -s -w "\n" -XPUT "${ELASTIC_ENDPOINT}/rdm/_settings" -d @/config/indices/rdm/analyzers.json
echo Open index rdm
curl -s -w "\n" -XPOST "${ELASTIC_ENDPOINT}/rdm/_open"
# Create collection version mapping by adding the parent field to the regular collection mapping json
cp -f /config/indices/rdm/mappings/collection.json /tmp/collection_version.json
sed -i '1s/.*/{"_parent": {"type": "collection"},/' /tmp/collection_version.json
echo Put collection version mapping
curl -s -w "\n" -XPUT "${ELASTIC_ENDPOINT}/rdm/_mapping/collection_version" -d @/tmp/collection_version.json
echo Put collection mapping
#curl -s -w "\n" -XPUT "${ELASTIC_ENDPOINT}/rdm/_mapping/collection_version" -d '{{"_parent": {"type": "collection"}}'
curl -s -w "\n" -XPUT "${ELASTIC_ENDPOINT}/rdm/_mapping/collection" -d @/config/indices/rdm/mappings/collection.json
echo Put user mapping
curl -s -w "\n" -XPUT "${ELASTIC_ENDPOINT}/rdm/_mapping/user" -d @/config/indices/rdm/mappings/user.json
}
COUNTER=1
while [ $COUNTER -lt 30 ]; do
if curl -s ${ELASTIC_ENDPOINT}/_cluster/health | grep -E "yellow|green" &> /dev/null; then
elasticsearch_post_startup
exit 0 &> /dev/null
else
let COUNTER=COUNTER+1
echo "Waiting for Elastic Search"
sleep 20
fi
done
echo Elastic did not start properly after 20 attempts. No post startup initialization performed.<file_sep>/mappings/create_index.sh
#!/bin/bash
if [ $# -ne 1 ]; then
echo "$0 <elasticIndexURL>"
echo
echo "example:"
echo
echo "$0 http://agaatvlinder.uci.ru.nl:9200/rdm"
exit 1
fi
URL_INDEX=$1
echo "DELETE $URL_INDEX ..."
curl -XDELETE $URL_INDEX
echo
echo "PUT $URL_INDEX ..."
curl -XPUT -H "Content-Type: application/json" -d @mapping.json $URL_INDEX
echo
| 9560db147adbb5b7a9a3a6bc83de6024a7631d3c | [
"Markdown",
"Shell"
] | 3 | Markdown | parallaxisjones/rdm-search-docker-compose | 37938511d5db09e30afad78638d150099db3bb65 | 75d84c902093dda2ec1dda3d8121fe665935cbe4 |
refs/heads/master | <repo_name>mrpcalcantara/MAXCTemplates<file_sep>/MAView.xctemplate/___FILEBASENAME___.swift
//
// ___FILENAME___
// ___PROJECTNAME___
//
// Created by ___FULLUSERNAME___ on ___DATE___.
// ___COPYRIGHT___
//
import UIKit
import Foundation
// MARK: - Protocols
class ___VARIABLE_productName___: UIView {
// MARK: - Properties
// MARK: - Subviews
// MARK: - Initializers
override init(frame: CGRect) {
super.init(frame: frame)
setup()
}
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
setup()
}
// MARK: - Functions
func setup() {
setupViews()
setupConstraints()
}
func setupViews() {
}
func setupConstraints() {
}
// MARK: - Private helper functions
}
// MARK: - Extensions
extension ___VARIABLE_productName___ {
}
| f3eb04fba0c1834c90353d434906bfb414aa45f8 | [
"Swift"
] | 1 | Swift | mrpcalcantara/MAXCTemplates | ae4661b0f1d0819558e1a5726b82d66043912d80 | bd29c16899b52921a001c87f3016d19dc6510722 |
refs/heads/main | <file_sep>package com.haibao;
/**
*
* 动态数组
* @author ml.c
* @date 19:01 PM 4/22/21
**/
public class Array<T> {
private T[] data;
private int size;
/**
* 有参构造
* @param capacity 初始容量
*/
public Array(int capacity){
data = (T[])new Object[capacity];
size = 0;
}
/**
* 无参构造
*/
public Array() {
this(100);
}
/**
* 获取数据中的元素个数
* @return
*/
public int getSize(){
return size;
}
/**
* 获取数组的容量
* @return
*/
public int getCapactiy(){
return data.length;
}
/**
* 判断数组是否为空
* @return
*/
public boolean isEmpty(){
return size == 0;
}
/**
* 向所有元素后添加一个新元素
* @param e 要添加的元素
*/
public void addLast(T e){
add(size,e);
}
/**
* 向所有元素前添加一个新元素
* @param e
*/
public void addFirst(T e){
add(0,e);
}
/**
* 在指定的第index个位置插入一个新元素e
* @param index
* @param e
*/
public void add(int index,T e){
if(size == data.length){
resize();
}
if(index < 0 || index > size){
// 扩容 或者 抛出异常
throw new IllegalArgumentException("add fail,index is Illegal");
}
for (int i = size - 1; i >= index ; i--) {
data[i + 1] = data[i];
}
data[index] = e;
size ++;
}
/**
* 查找是否存在元素e
* @param e
* @return true / false
*/
public boolean contains(T e){
for (int i = 0; i < size; i++) {
if(data[i] == e){
return true;
}
}
return false;
}
public T get(int index){
if(index < 0 || index >= size){
throw new IllegalArgumentException("Get faild. Index is Illegal.");
}
return data[index];
}
public T getFirst(){
return get(0);
}
public T getLast(){
return get(size -1);
}
/**
* 查找是否存在元素e,并返回元素e的索引
* 如果不存在,返回-1
* @param e
* @return 索引位置
*/
public int find(T e){
for (int i = 0; i < size; i++) {
if (data[i] == e){
return i;
}
}
return -1;
}
/**
* 查找所有元素e,并返回所有索引
* @param e
* @return
*/
public int[] findAll(T e){
int[] indexs = new int[size];
int j = 0;
for (int i = 0; i < size; i++) {
if (data[i] == e){
indexs[j] = i;
j++;
}
}
return indexs;
}
/**
* 根据索引,删除 元素,并返回元素
* @param index
* @return
*/
public T remove(int index){
if(index < 0 || index > size){
throw new IllegalArgumentException("remove failed.Index is Illegal");
}
T ret = data[index];
for (int i = index + 1; i < size; i++) {
data[i - 1] = data[i];
}
size --;
//loitering objects 闲散对象,等待GC
data[size] = null;
//缩容
//防止复杂度的震荡 lazy
if(size < data.length / 4 && data.length / 2 != 0){
resize(data.length / 2);
}
return ret;
}
/**
* 删除第一个索引,返回删除的元素
* @return
*/
public T removeFirst(){
return this.remove(0);
}
/**
* 删除最后一个索引,返回删除的元素
* @return
*/
public T removeLast(){
return this.remove(size - 1);
}
/**
* 删除一个元素e
* @param e
*/
public boolean removeElement(T e){
int index = find(e);
if(index != -1){
remove(index);
return true;
}
return false;
}
/**
* 删除所有元素e
* @param e
* @return
*/
public boolean removeAllElement(T e){
int[] indexs = findAll(e);
if(indexs.length > 0 ){
for (int i = 0; i < indexs.length; i++) {
remove(indexs[i]);
}
return true;
}
return false;
}
/**
* 默认 扩容 2倍
*/
private void resize(){
resize(getCapactiy() * 2);
}
/**
* 扩容,指定 容量
* @param newCapactiy
*/
private void resize(int newCapactiy){
T[] newData = (T[])new Object[newCapactiy];
for (int i = 0; i < size; i++) {
newData[i] = data[i];
}
data = newData;
}
@Override
public String toString() {
StringBuilder res = new StringBuilder();
res.append(String.format("Array, size = %d, capacity = %d \n", size, data.length));
res.append("[");
for (int i = 0; i < size; i++) {
res.append(data[i]);
if(i != size - 1){
res.append(",");
}
}
res.append("]");
return res.toString();
}
}
<file_sep>package com.haibao;
import org.junit.jupiter.api.Test;
/**
* 测试
*
* @author ml.c
* @date 19:07 PM 4/22/21
**/
public class LocalTest {
/**
* 测试 数组栈
*/
@Test
public void testArrayStack(){
ArrayStack<Integer> arrayStack = new ArrayStack();
for (int i = 0; i < 5; i++) {
arrayStack.push(i);
}
System.out.println(arrayStack.toString());
int size = arrayStack.getSize();
for (int i = 0; i < size; i++) {
arrayStack.pop();
System.out.println(arrayStack.toString());
}
}
/**
* 测试数组
*/
@Test
public void testArray(){
Array array = new Array(20);
for (int i = 0; i < 10; i++) {
array.addLast(i);
}
System.out.println(array.toString());
array.add(1,100);
System.out.println(array.toString());
int e = array.find(100);
System.out.println(e);
array.remove(2);
array.removeElement(100);
System.out.println(array.toString());
array.add(1,100);
array.add(2,100);
System.out.println(array.toString());
array.removeAllElement(100);
System.out.println(array.toString());
for (int i = 0; i < 100; i++) {
array.addLast(i);
}
System.out.println(array.toString());
for (int i = 99; i > 40; i--) {
array.remove(i);
}
System.out.println(array.toString());
}
}
<file_sep># 栈 LIFO
last in first out
## 应用场景
- UNDO 操作
- 程序调用的系统栈<file_sep># 结构说明
- Data-Structure 数据结构
- Algorithm 算法
- LeetCode 题库
# 数据结构 笔记
1.2 [栈](./Data-Structure/doc/LIFO.md)
| 464b35c04a820d77f2dbc9077f60cbce4091204f | [
"Markdown",
"Java"
] | 4 | Java | wuquegongzi/Algorithm-in-Practice | 4a3e214c09d0f7e66d10d9258d14f343816551ee | fe42e25c8cda00c78faa4c97a38ae5f058a44ef9 |
refs/heads/master | <repo_name>wycliffkas/NoteKeeper<file_sep>/README.md
## NoteKeeper
Minimal & elegant note keeper app using SQLite as a DB.
<p float="left">
<img src="https://github.com/wycliffkas/Diary/blob/master/screen.png" width="215" height="400" />
<img src="https://github.com/wycliffkas/Diary/blob/master/screen2.png" width="215" height="400" />
<img src="https://github.com/wycliffkas/Diary/blob/master/screen3.png" width="215" height="400" />
<img src="https://github.com/wycliffkas/Diary/blob/master/screen4.png" width="215" height="400" />
</p>
### Features
* View notes and courses
* Add notes
* Share notes
* update a notes
### How to use
To clone and run this repository you'll need Git installed on your computer. From your command line:
```bash
# Clone this repository
* https://github.com/wycliffkas/NoteKeeper.git
# Go into the repository
* cd NoteKeeper
* Open the application in Android Studio
* Run the Application
```
<file_sep>/app/src/main/java/com/example/notekeeper/CourseRecyclerAdapter.kt
package com.example.notekeeper
import android.content.Context
import android.content.Intent
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.TextView
import androidx.recyclerview.widget.RecyclerView
class CourseRecyclerAdapter(private val context: Context, private val courses: List<CourseInfo>) :
RecyclerView.Adapter<CourseRecyclerAdapter.ViewHolder>() {
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val itemView = layoutInflater.inflate(R.layout.item_course_list, parent, false)
return ViewHolder(itemView)
}
private val layoutInflater = LayoutInflater.from(context)
override fun getItemCount() = courses.size
override fun onBindViewHolder(holder: CourseRecyclerAdapter.ViewHolder, position: Int) {
val course = courses[position]
holder.title?.text = course.title
}
inner class ViewHolder(itemView: View?) : RecyclerView.ViewHolder(itemView!!) {
val title = itemView?.findViewById<TextView?>(R.id.courseTitle)
}
} | 962b85da53f7e9f21542732ae2e873bc61566a85 | [
"Markdown",
"Kotlin"
] | 2 | Markdown | wycliffkas/NoteKeeper | 286f7757eca1c734a0ca321b7c08f92f9469b0ac | f690b9038d7a4594f087aa9d552a24a82075c1ee |
refs/heads/master | <file_sep>using System.Collections.Generic;
using UnityEngine;
using UnityEditor;
using System.IO;
public class Blocks : MonoBehaviour {
public Dictionary<string, int> blocks = new Dictionary<string, int>();
void Start() {
UpdateDictionary();
}
public void UpdateDictionary(bool writeToTxt = false, string contentName = " ", string contentId = " ") {
TextAsset txt = (TextAsset)Resources.Load("blocks", typeof(TextAsset));
List<string> lines = new List<string>(txt.text.Split('\n'));
blocks = new Dictionary<string, int>();
if (writeToTxt) {
WriteToTxt(contentName, contentId);
}
foreach (string line in lines) {
string name = line.Substring(0, line.IndexOf(" "));
int id = int.Parse(line.Substring(line.IndexOf(" ")));
blocks.Add(name, id);
}
}
void WriteToTxt(string name, string id) {
string path = "Assets/Resources/blocks.txt";
StreamWriter writer = new StreamWriter(path, true);
writer.Write(System.Environment.NewLine + name + " " + id);
writer.Close();
AssetDatabase.ImportAsset(path);
}
public string GetName(int id) {
foreach (KeyValuePair<string, int> kv in blocks) {
if (kv.Value == id) {
return kv.Key;
}
}
return "";
}
}
<file_sep># InGameContentEditor
In game content editor for blocks, items and mobs

<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Block : MonoBehaviour {
public Blocks blocks;
public Sprite sprite;
public string name = "";
public int ID;
public float hardness = 3;
private void OnMouseEnter() {
GetComponent<SpriteRenderer>().color = new Color(.5f, .5f, .5f);
}
private void OnMouseExit() {
GetComponent<SpriteRenderer>().color = new Color(1, 1, 1);
}
private void OnMouseOver() {
if (Input.GetMouseButton(0) && hardness >= 0) {
hardness -= Time.deltaTime;
}
if (hardness <= 0) {
//Add to inventory
Destroy(this.gameObject);
}
}
public void SetSprite() {
sprite = Resources.Load<Sprite>(ID.ToString());
GetComponent<SpriteRenderer>().sprite = sprite;
}
public void SetName() {
blocks = GameObject.FindGameObjectWithTag("Blocks").GetComponent<Blocks>();
name = blocks.GetName(ID);
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using System.IO;
using UnityEngine;
using UnityEditor;
using TMPro;
public class BlockEditor : MonoBehaviour {
public GameObject blockEditor;
public GameObject sprite;
public Sprite saveHover;
public Sprite browseHover;
public SpriteRenderer saveSR;
public SpriteRenderer browseSR;
public TextMeshProUGUI NAME;
public TextMeshProUGUI ID;
public Texture2D texture;
public Blocks blocks;
byte[] fileContent;
int id = 0;
void Start() {
blockEditor.SetActive(false);
}
void Update() {
GetMouseInfo();
//FOR VISAUL DEMONSTRATION ONLY (GONNA REMOVE EVERYTHING UNDERNEATH) \/
//
}
void Browse() {
string path = EditorUtility.OpenFilePanel("Block Editor", "", "png");
if (path.Length != 0) {
fileContent = File.ReadAllBytes(path);
Debug.Log(path);
Texture2D tex = new Texture2D(8, 8, TextureFormat.PVRTC_RGBA4, false);
tex.filterMode = FilterMode.Point;
tex.LoadImage(fileContent);
tex.Apply();
sprite.GetComponent<SpriteRenderer>().sprite = Sprite.Create(tex, new Rect(0.0f, 0.0f, tex.width, tex.height), new Vector2(0.5f, 0.5f), 8);
NAME.text = GetNameFromPath(path, '/');
id = GetID(@"C:\Users\isak.horvath\Documents\Console\Assets\Resources\");
ID.text = id.ToString();
}
}
int GetID(string path) {
return DirCount(new DirectoryInfo(path));
}
string GetNameFromPath(string input, char flag) {
string[] temp = input.Split(flag);
string output = temp[temp.Length - 1];
int index = output.LastIndexOf(".");
if (index > 0) {
output = output.Substring(0, index);
}
return output;
}
public int DirCount(DirectoryInfo d) {
int i = 0;
FileInfo[] fis = d.GetFiles();
foreach (FileInfo fi in fis) {
if (fi.Extension.Contains("png")) {
i++;
}
}
return i;
}
void Save() {
File.WriteAllBytes(@"C:\Users\isak.horvath\Documents\Console\Assets\Resources\" + id + ".png", fileContent);
blocks.UpdateDictionary(true, NAME.text, id.ToString());
Debug.Log("Saved File...");
}
void GetMouseInfo() {
RaycastHit2D hit = Physics2D.Raycast(Camera.main.ScreenToWorldPoint(Input.mousePosition), Vector2.zero);
if (hit.collider != null) {
//BLOCK EDITOR BUTTON
if (hit.collider.name == "Block_Editor_Button") {
if (Input.GetMouseButtonUp(0)) {
blockEditor.SetActive(true);
}
}
//BROWSE
if (hit.collider.name == "Brows") {
browseSR.sprite = browseHover;
if (Input.GetMouseButtonUp(0)) {
Browse();
}
}
else {
browseSR.sprite = null;
}
//CLOSE
if (hit.collider.name == "Close") {
if (Input.GetMouseButtonUp(0)) {
blockEditor.SetActive(false);
}
}
//SAVE
if (hit.collider.name == "Save") {
saveSR.sprite = saveHover;
if (Input.GetMouseButtonUp(0)) {
Save();
}
}
else {
saveSR.sprite = null;
}
}
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Spawner : MonoBehaviour {
public GameObject sprite;
void Start() {
Render();
}
void Render() {
for (int y = 0; y < 50; y++) {
for (int x = 0; x < 100; x++) {
GameObject temp = Instantiate(sprite, new Vector3(transform.position.x + x, transform.position.y - y, transform.position.z), Quaternion.identity);
if (transform.position.y - y < 19.5f && transform.position.y - y > 16.5f) {
temp.GetComponent<Block>().ID = 0;
}
else if (transform.position.y - y <= 16.5) {
temp.GetComponent<Block>().ID = 1;
}
else {
temp.GetComponent<Block>().ID = 2;
}
temp.GetComponent<Block>().SetSprite();
temp.GetComponent<Block>().SetName();
}
}
}
}
| f16cb7d9f8897ca5e6726ffbcad46c9adf3d4419 | [
"Markdown",
"C#"
] | 5 | C# | tavro/InGameContentEditor | 4dd7c67aaf368913d3a7f65a9376f0f847a546e8 | d5bb1323855dd039d9054929c8b78dda5b0330d9 |
refs/heads/master | <repo_name>SAndroidEOfficial/raspberry-ble<file_sep>/gpio_oldV01.js
var util = require('util');
var events = require('events');
var GPIOmode = {
NONE: -1,
INPUT: 0,
OUTPUT: 1,
};
// GPIO.prototype.getIOGPIOmodeINPUT=function(){
// return GPIOmode.INPUT;
// }
// GPIO.prototype.getIOGPIOmodeNONE=function(){
// return GPIOmode.NONE;
// }
// GPIO.prototype.getIOGPIOmodeOUTPUT=function(){
// return GPIOmode.OUTPUT;
// }
var GPIOstatus = {
LOW: 0,
HIGH: 1,
}
var Gpio = require('../node_modules/onoff').Gpio, // Constructor function for Gpio objects.
io,
iv,
_updateValueCallback;
var GpioNum;
var me;
function GPIO(num) {
this.GpioNum=num;
console.log('gpio - new Gpio n: '+this.GpioNum);
this.io = new Gpio(num, 'in', 'both');
me=this;
/////
this.io.watch(function (err, value) {
if (err) {
throw err;
}
console.log('constr: callback is '+this+' '+this.GpioNum);
if (me._updateValueCallback!=null){
me._updateValueCallback(new Buffer([value]));
}
me.IOstatus = value;
});
console.log('direction is: '+this.io.direction());
/////
events.EventEmitter.call(this);
this.IOmode = GPIOmode.INPUT;
this.IOstatus = GPIOstatus.LOW;
this._updateValueCallback = null;
this.getIOGPIOmodeINPUT=function(){
return GPIOmode.INPUT;
}
this.getIOGPIOmodeNONE=function(){
return GPIOmode.NONE;
}
this.getIOGPIOmodeOUTPUT=function(){
return GPIOmode.OUTPUT;
}
//this._value = new Buffer(0);
}
module.exports.GPIO = GPIO;
util.inherits(GPIO, events.EventEmitter);
GPIO.prototype.setMode = function(mode) {
console.log('gpio - set mode: '+mode+" to Gpio n: "+this.GpioNum);
if (mode==GPIOmode.INPUT){
//io.unexport();
if (me.IOmode!=GPIOmode.INPUT){
me.IOmode=GPIOmode.INPUT;
this.io.setDirection('in');
this.io.setEdge('both');
this.io.watch(function (err, value) {
if (err) {
throw err;
}
//callback=this.getCallback();
console.log('input: callback is '+this+' '+this.GpioNum);
if (me._updateValueCallback!=null){
//console.log('callback not null ');
me._updateValueCallback(new Buffer([value]));
}
me.IOstatus = value;
//console.log('Button pressed!, its value was ' + value);
});
}
}else if(mode==GPIOmode.OUTPUT){
if (me.IOmode!=GPIOmode.OUTPUT){
this.IOmode=GPIOmode.OUTPUT;
console.log('direction is: '+this.io.direction());
this.io.setEdge('none');
this.io.unwatch();
var delay=100; //1 second
setTimeout(function() {
this.io.setDirection('out');
}, delay);
setTimeout(function() {
console.log('direction is: '+this.io.direction());
if(this.io.readSync()!=0){
this.io.writeSync(0);
}
}, delay*2);
//
//io = Gpio(this.GpioNum, 'out');
}
}
};
GPIO.prototype.setStatus=function(value){
console.log('writing value'+" to Gpio n: "+this.GpioNum);
if (this.IOmode==GPIOmode.OUTPUT){
console.log('writing value: '+value);
this.io.writeSync(value);
}
}
GPIO.prototype.readIOstatus=function(){
this.IOstatus = this.io.readSync();
return this.IOstatus;
}
GPIO.prototype.setCallbackFunct=function(callback){
this._updateValueCallback = callback;
console.log('updated callback');
}
GPIO.prototype.getGPIOMODE=function(mode){
if (mode==GPIOmode.OUTPUT)
return GPIOmode.OUTPUT;
else if (mode==GPIOmode.INPUT)
return GPIOmode.INPUT;
else
return GPIOmode.NONE
}
GPIO.prototype.getGPIOMODE_OUTPUT=function(){
return GPIOmode.OUTPUT;
}
GPIO.prototype.getGPIOMODE_INPUT=function(){
return GPIOmode.INPUT;
}
//test
/* GPIO.prototype.notificationTest=function(){
if (this._updateValueCallback==null){
console.log('gpio - updateValueCallback è null');
}
io.unexport();
me.IOmode=GPIOmode.INPUT;
io = new Gpio(14, 'in', 'both');
io.watch(function (err, value) {
if (err) {
throw err;
}
//callback=this.getCallback();
console.log('callback is '+me);
if (me._updateValueCallback!=null){
//console.log('callback not null ');
me._updateValueCallback(new Buffer([value]));
}
me.IOstatus = value;
//console.log('Button pressed!, its value was ' + value);
});
} */
<file_sep>/gpio-serviceV01.js
var util = require('util');
var bleno = require('../node_modules/bleno');
var GpioOutputCharacteristic = require('./gpio-output-characteristic');
var GpioModeCharacteristic = require('./gpio-mode-characteristic');
var GpioInputCharacteristic = require('./gpio-input-characteristic');
function GPIOService(gpio, uuidMine, uuidMode, uuidIn, uuidOut) {
bleno.PrimaryService.call(this, {
uuid: uuidMine,
characteristics: [
new GpioModeCharacteristic(gpio, uuidMode),
new GpioOutputCharacteristic(gpio, uuidOut),
new GpioInputCharacteristic(gpio, uuidIn)
]
});
}
util.inherits(GPIOService, bleno.PrimaryService);
module.exports = GPIOService;
<file_sep>/gpio-mode-characteristic_errV01.js
var util = require('util');
var bleno = require('../node_modules/bleno');
var gpio = require('./gpio');
function GpioSetModeCharacteristic(gpio, uuidMine) {
bleno.Characteristic.call(this,{
uuid: uuidMine,
properties: ['read', 'write'],
descriptors:[
new bleno.Descriptor({
uuid: '2901',
value: 'Gets or sets the mode of the GPIO.'
})
]
});
this.gpio = gpio;
this.onWriteRequestMe = function(data, offset, withoutResponse, callback){
console.log('GpioSetModeCharacteristic - Write request');
if (offset) {
callback(this.RESULT_ATTR_NOT_LONG);
}
else if (data.length !== 1) {
callback(this.RESULT_INVALID_ATTRIBUTE_LENGTH);
}
else{
var mode = data.readUInt8(0);
console.log('GpioSetModeCharacteristic - Write request: data: '+data);
console.log('GpioSetModeCharacteristic - Write request: mode: '+mode);
switch (mode) {
case this.gpio.getIOGPIOmodeINPUT():
case this.gpio.getIOGPIOmodeNONE():
case this.gpio.getIOGPIOmodeOUTPUT():
this.gpio.setMode(mode);
callback(this.RESULT_SUCCESS);
break;
default:
callback(this.RESULT_UNLIKELY_ERROR);
break;
}
}
};
this.onReadRequestMe = function(offset, callback){
console.log('GpioSetModeCharacteristic - Read request');
if (offset) {
callback(this.RESULT_ATTR_NOT_LONG, null);
}
else {
var data = new Buffer(1);
data.writeUInt8(this.gpio.IOmode, 0);
callback(this.RESULT_SUCCESS, data);
}
};
me=this;
}
util.inherits(GpioSetModeCharacteristic, bleno.Characteristic);
GpioSetModeCharacteristic.prototype.onWriteRequest = function(data, offset, withoutResponse, callback) {
console.log('GpioSetModeCharacteristic - Write request');
me.onWriteRequestMe(data, offset, withoutResponse, callback);
};
GpioSetModeCharacteristic.prototype.onReadRequest = function(offset, callback) {
console.log('GpioSetModeCharacteristic - Read request');
me.onReadRequestMe(offset, callback);
};
module.exports = GpioSetModeCharacteristic;<file_sep>/gpio-input-characteristicV01.js
var util = require('util');
var bleno = require('../node_modules/bleno');
var gpio = require('./gpio');
function GpioInputCharacteristic(gpio, uuidMine) {
bleno.Characteristic.call(this, {
uuid: uuidMine,
properties: ['read', 'write', 'notify'],
descriptors: [
new bleno.Descriptor({
uuid: '2903',
value: 'read the vslue of the GPIO.'
})
]
});
this.gpio = gpio;
}
util.inherits(GpioInputCharacteristic, bleno.Characteristic);
GpioInputCharacteristic.prototype.onReadRequest = function(offset, callback) {
console.log('GpioInputCharacteristic - Read request');
if (offset) {
callback(this.RESULT_ATTR_NOT_LONG, null);
}
else{
var data = new Buffer(1);
data.writeUInt8(this.gpio.readIOstatus(), 0);
callback(this.RESULT_SUCCESS, data);
}
};
GpioInputCharacteristic.prototype.onSubscribe = function(maxValueSize, updateValueCallback) {
console.log('GpioInputCharacteristic - onSubscribe');
console.log('maxValuesize: '+maxValueSize);
if (updateValueCallback==null){
console.log('updateValueCallback è null');
}
this.gpio.setCallbackFunct(updateValueCallback);
};
GpioInputCharacteristic.prototype.onUnsubscribe = function() {
console.log('GpioInputCharacteristic - onUnsubscribe');
this.gpio._updateValueCallback = null;
};
module.exports = GpioInputCharacteristic;<file_sep>/README.md
# raspberry-ble
Javascript Bluetooth scripts to be installed on Raspberry to make it compatible with SAndroidE
Work in progress!
Code based on the library https://github.com/sandeepmistry/bleno
Tested and working on Raspberry Pi 3, see tutorial at link https://github.com/SAndroidEOfficial/framework/wiki/T1-Arduino-and-2-RaspBerry
<file_sep>/gpio_buV01.js
var util = require('util');
var events = require('events');
var GPIOmode = {
NONE: 0,
INPUT: 1,
OUTPUT: 2,
};
var GPIOstatus = {
LOW: 0,
HIGH: 1,
}
var Gpio = require('../node_modules/onoff').Gpio, // Constructor function for Gpio objects.
io = new Gpio(14, 'in'),
iv;
function GPIO() {
events.EventEmitter.call(this);
this.IOmode = GPIOmode.NONE;
this.IOstatus = GPIOstatus.LOW;
this._updateValueCallback = null;
}
util.inherits(GPIO, events.EventEmitter);
GPIO.prototype.setMode = function(mode) {
if (mode==GPIOmode.INPUT){
io.unexport();
this.IOmode=GPIOmode.INPUT;
io = new Gpio(14, 'in', 'both');
io.watch(function (err, value) {
if (err) {
throw err;
}
if (_updateValueCallback!=null){
this.IOstatus = value;
this._updateValueCallback(value);
}
console.log('Button pressed!, its value was ' + value);
});
}else if(mode==GPIOmode.OUTPUT){
io.unexport();
this._updateValueCallback=null;
this.IOmode=GPIOmode.OUTPUT;
io = Gpio(14, 'out');
if(io.readSync()!=0){
io.writeSync(0);
}
}
};
GPIO.prototype.seStatus=function(value){
if (this.IOmode==GPIOmode.OUTPUT){
io.writeSync(value);
}
}
GPIO.prototype.readIOstatus=function(){
this.IOstatus = io.readSync();
}
module.exports.GPIO = GPIO;
<file_sep>/gpio-output-characteristicV01.js
var util = require('util');
var bleno = require('../node_modules/bleno');
function GpioSetOutputCharacteristic(gpio, uuidMine) {
bleno.Characteristic.call(this,{
uuid: uuidMine,
properties: ['read', 'write'],
descriptors: [
new bleno.Descriptor({
uuid: '2902',
value: 'sets the vslue of the GPIO.'
})
]
});
this.gpio = gpio;
}
util.inherits(GpioSetOutputCharacteristic, bleno.Characteristic);
GpioSetOutputCharacteristic.prototype.onWriteRequest = function(data, offset, withoutResponse, callback) {
console.log('GpioSetOutputCharacteristic - Write request');
if (offset) {
callback(this.RESULT_ATTR_NOT_LONG);
}
else if (data.length !== 1) {
callback(this.RESULT_INVALID_ATTRIBUTE_LENGTH);
}
else {
console.log('GpioSetOutputCharacteristic - Write request: in write else');
console.log('GpioSetOutputCharacteristic - Write request: this.gpio.IOmode: '+this.gpio.IOmode);
console.log('GpioSetOutputCharacteristic - Write request: this.gpio.IOmode: '+this.gpio.getIOGPIOmodeOUTPUT());
if (this.gpio.IOmode==this.gpio.getIOGPIOmodeOUTPUT()){
var value = data.readUInt8(0);
console.log('GpioSetOutputCharacteristic - Write request: setting value: '+value);
switch (value) {
case 0:
case 1:
console.log('GpioSetOutputCharacteristic - Write request: setting value: '+value);
this.gpio.setStatus(value);
callback(this.RESULT_SUCCESS);
break;
default:
callback(this.RESULT_UNLIKELY_ERROR);
break;
}
}
else{
callback(this.RESULT_UNLIKELY_ERROR);
}
}
};
GpioSetOutputCharacteristic.prototype.onReadRequest = function(offset, callback) {
console.log('GpioSetOutputCharacteristic - Read request');
if (offset) {
callback(this.RESULT_ATTR_NOT_LONG, null);
}
else {
var data = new Buffer(1);
this.gpio.readIOstatus();
data.writeUInt8(this.gpio.IOstatus, 0);
callback(this.RESULT_SUCCESS, data);
}
};
module.exports = GpioSetOutputCharacteristic;<file_sep>/gpioV01.js
var util = require('util');
var events = require('events');
var bleno = require('../node_modules/bleno');
var BlenoCharacteristic = bleno.Characteristic;
var GPIOmode = {
NONE: -1,
INPUT: 0,
OUTPUT: 1,
};
var GPIOstatus = {
LOW: 0,
HIGH: 1,
}
// Constructor function for Gpio objects.
var Gpio = require('../node_modules/onoff').Gpio,io,iv,_updateValueCallback;
var GpioNum;
var me;
var gpioValue=0;
var setDir = function(eo){
eo.io.setDirection('out');
};
var setVaue = function(eo){
if(eo.io.readSync()!=0){
eo.io.writeSync(0);}
};
var setCB = function(eo){
eo.io.watch(function(err, value){
if (err) {
throw err;
}
console.log('input: callback is '+eo+' '+eo.GpioNum+', real gpio: '+eo.io.gpio);
if (eo._updateValueCallback!=null){
console.log('input: callo, value= '+value);
if (eo.gpioValue!=value){
eo.gpioValue=value;
eo._updateValueCallback(new Buffer([value]));
}
}
eo.IOstatus = value;
});
}
function GPIO(num) {
this.GpioNum=num;
this.io = new Gpio(num, 'in', 'both');
console.log('gpio - new Gpio n: '+this.GpioNum+', real gpio: '+this.io.gpio);
setCB(this);
console.log('direction is: '+this.io.direction());
events.EventEmitter.call(this);
this.IOmode = GPIOmode.INPUT;
this.IOstatus = GPIOstatus.LOW;
this._updateValueCallback = null;
me=this;
this.getIOGPIOmodeINPUT=function(){
return GPIOmode.INPUT;
}
this.getIOGPIOmodeNONE=function(){
return GPIOmode.NONE;
}
this.getIOGPIOmodeOUTPUT=function(){
return GPIOmode.OUTPUT;
}
this.setMode = function(mode) {
console.log('gpio - set mode: '+mode+" to Gpio n: "+this.GpioNum+', real gpio: '+this.io.gpio);
if (mode==GPIOmode.INPUT){
if (me.IOmode!=GPIOmode.INPUT){
me.IOmode=GPIOmode.INPUT;
this.io.setDirection('in');
this.io.setEdge('both');
setCB(this);
}
}else if(mode==GPIOmode.OUTPUT){
if (me.IOmode!=GPIOmode.OUTPUT){
this.IOmode=GPIOmode.OUTPUT;
console.log('direction is: '+this.io.direction()+', real gpio: '+this.io.gpio);
this.io.setEdge('none');
this.io.unwatch(this.callbackInput);
var delay=100; //1 second
setTimeout(setDir(this),delay);
setTimeout(setVaue(this),delay*2);
}
}
};
this.setStatus=function(value){
console.log('writing value'+" to Gpio n: "+this.GpioNum+', real gpio: '+this.io.gpio);
if (this.IOmode==GPIOmode.OUTPUT){
console.log('writing value: '+value);
this.io.writeSync(value);
}
};
this.readIOstatus=function(){
this.IOstatus = this.io.readSync();
return this.IOstatus;
};
this.setCallbackFunct=function(callback){
this._updateValueCallback = callback;
console.log('updated callback');
};
}
exports.GPIO = GPIO;
util.inherits(GPIO, events.EventEmitter);
GPIO.prototype.getGPIOMODE=function(mode){
if (mode==GPIOmode.OUTPUT)
return GPIOmode.OUTPUT;
else if (mode==GPIOmode.INPUT)
return GPIOmode.INPUT;
else
return GPIOmode.NONE
}
GPIO.prototype.getGPIOMODE_OUTPUT=function(){
return GPIOmode.OUTPUT;
}
GPIO.prototype.getGPIOMODE_INPUT=function(){
return GPIOmode.INPUT;
} | 75e8fe205bcbe98f151d48ff2de793347a786825 | [
"JavaScript",
"Markdown"
] | 8 | JavaScript | SAndroidEOfficial/raspberry-ble | 7c7ba8fbfaa80dab79425873399315d06d1349cf | 5d4028ce7f153e2ac7d68f60b14b2e6df4e021b8 |
refs/heads/master | <file_sep>// Project: Daggerfall Tools For Unity
// Copyright: Copyright (C) 2009-2016 Daggerfall Workshop
// Web Site: http://www.dfworkshop.net
// License: MIT License (http://www.opensource.org/licenses/mit-license.php)
// Source Code: https://github.com/Interkarma/daggerfall-unity
// Original Author: TheLacus
// Contributors:
//
// Notes: Uses AssetBundle instead of www.movie because of this:
// (https://issuetracker.unity3d.com/issues/calling-www-dot-movie-fails-to-load-and-prints-error-loadmovedata-got-null)
//
using System.IO;
using UnityEngine;
namespace DaggerfallWorkshop.Utility.AssetInjection
{
/// <summary>
/// Handles import and injection of custom videos
/// with the purpose of providing modding support.
/// </summary>
static public class VideoReplacement
{
static public string videosPath = Path.Combine(Application.streamingAssetsPath, "Videos");
const string extension = ".dfvideo";
const string fileExtension = ".ogg";
/// <summary>
/// Check if video file exist on disk.
/// </summary>
/// <param name="name">Name of sound file.</param>
static public bool CustomVideoExist(string name)
{
name = name.Replace(".VID", extension);
if (DaggerfallUnity.Settings.MeshAndTextureReplacement
&& File.Exists(Path.Combine(videosPath, name)))
return true;
return false;
}
/// <summary>
/// Get Video.
/// </summary>
/// <param name="name">Name of sound file.</param>
/// <returns>New WWW object</returns>
static public MovieTexture GetVideo(string name)
{
// Get AssetBundle
name = name.Replace(".VID", extension);
string path = Path.Combine(videosPath, name);
var loadedAssetBundle = AssetBundle.LoadFromFile(path);
// Get MovieTexture
name = name.Replace(extension, fileExtension);
if ((loadedAssetBundle != null) && (loadedAssetBundle.Contains(name)))
return loadedAssetBundle.LoadAsset<MovieTexture>(name);
Debug.LogError(string.Format("File {0} from {1} is corrupted", name, videosPath));
return null;
}
}
}
<file_sep>// Project: Daggerfall Tools For Unity
// Copyright: Copyright (C) 2009-2016 Daggerfall Workshop
// Web Site: http://www.dfworkshop.net
// License: MIT License (http://www.opensource.org/licenses/mit-license.php)
// Source Code: https://github.com/Interkarma/daggerfall-unity
// Original Author: Uncanny_Valley
// Contributors: TheLacus
//
// Notes:
//
/*
* TODO:
* - StreamingWorld
* - Optimize FinaliseCustomGameObject() to avoid importing the same texture more than once
*/
using System.IO;
using UnityEngine;
using DaggerfallWorkshop.Game;
using DaggerfallWorkshop.Game.Utility.ModSupport;
namespace DaggerfallWorkshop.Utility.AssetInjection
{
/// <summary>
/// Handles import and injection of custom meshes and materials
/// with the purpose of providing modding support.
/// </summary>
static public class MeshReplacement
{
#region Fields
// Paths
static public string modelsPath = Path.Combine(Application.streamingAssetsPath, "Models");
static public string flatsPath = Path.Combine(Application.streamingAssetsPath, "Flats");
// Extensions
const string modelExtension = ".model";
const string flatExtension = ".flat";
// Variants
const string winterTag = "_winter";
#endregion
#region Models
/// <summary>
/// Import the custom GameObject if available
/// </summary>
/// <returns>Returns the imported model or null.</returns>
static public GameObject ImportCustomGameobject (uint modelID, Vector3 position, Transform parent, Quaternion rotation)
{
// Check user settings
if (!DaggerfallUnity.Settings.MeshAndTextureReplacement)
return null;
// Import Gameobject from Resources
// This is useful to test models
if (ReplacementPrefabExist(modelID))
return LoadReplacementPrefab(modelID, position, parent, rotation);
// Get model from disk
string modelName = modelID.ToString();
string path = Path.Combine(modelsPath, modelName + modelExtension);
if (File.Exists(path))
{
// Get AssetBundle
AssetBundle LoadedAssetBundle;
if (TryGetAssetBundle(path, modelName, out LoadedAssetBundle))
{
// Assign the name according to the current season
if (IsWinter())
{
if (LoadedAssetBundle.Contains(modelName + winterTag))
modelName += winterTag;
}
// Instantiate GameObject
GameObject object3D = GameObject.Instantiate(LoadedAssetBundle.LoadAsset<GameObject>(modelName));
LoadedAssetBundle.Unload(false);
InstantiateCustomModel(object3D, ref position, parent, ref rotation, modelName);
return object3D;
}
}
// Get model from mods using load order
Mod[] mods = ModManager.Instance.GetAllMods(true);
for (int i = mods.Length; i-- > 0;)
{
if (mods[i].AssetBundle.Contains(modelName))
{
// Assign the name according to the current season
if (IsWinter())
{
if (mods[i].AssetBundle.Contains(modelName + winterTag))
modelName += winterTag;
}
GameObject go = mods[i].GetAsset<GameObject>(modelName, true);
if (go != null)
{
InstantiateCustomModel(go, ref position, parent, ref rotation, modelName);
return go;
}
Debug.LogError("Failed to import " + modelName + " from " + mods[i].Title + " as GameObject.");
}
}
return null;
}
/// <summary>
/// Check existence of gameobject in Resources
/// </summary>
/// <returns>Bool</returns>
static public bool ReplacementPrefabExist(uint modelID)
{
if (DaggerfallUnity.Settings.MeshAndTextureReplacement //check .ini setting
&& Resources.Load("Models/" + modelID.ToString() + "/" + modelID.ToString()) != null)
return true;
return false;
}
/// <summary>
/// Import gameobject from Resources
/// </summary>
static public GameObject LoadReplacementPrefab(uint modelID, Vector3 position, Transform parent, Quaternion rotation)
{
GameObject object3D = null;
string name = modelID.ToString();
string path = "Models/" + name + "/";
// Assign the name according to the current season
if (IsWinter())
{
if (Resources.Load(path + name + winterTag) != null)
name += winterTag;
}
// Import GameObject
object3D = GameObject.Instantiate(Resources.Load(path + name) as GameObject);
InstantiateCustomModel(object3D, ref position, parent, ref rotation, modelID.ToString());
return object3D;
}
#endregion
#region Flats
/// <summary>
/// Import the custom GameObject for billboard if available
/// </summary>
/// <param name="inDungeon">Fix position for dungeon models.</param>
/// <returns>Returns the imported model or null.</returns>
static public GameObject ImportCustomFlatGameobject (int archive, int record, Vector3 position, Transform parent, bool inDungeon = false)
{
// Check user settings
if (!DaggerfallUnity.Settings.MeshAndTextureReplacement)
return null;
// Import Gameobject from Resources
// This is useful to test models
if (ReplacementFlatExist(archive, record))
return LoadReplacementFlat(archive, record, position, parent, inDungeon);
// Get model from disk
string modelName = archive.ToString() + "_" + record.ToString();
string path = Path.Combine(flatsPath, modelName + flatExtension);
if (File.Exists(path))
{
// Get AssetBundle
AssetBundle LoadedAssetBundle;
if (TryGetAssetBundle(path, modelName, out LoadedAssetBundle))
{
// Instantiate GameObject
GameObject go = GameObject.Instantiate(LoadedAssetBundle.LoadAsset<GameObject>(modelName));
LoadedAssetBundle.Unload(false);
InstantiateCustomFlat(go, ref position, parent, archive, record, inDungeon);
return go;
}
}
// Get model from mods using load order
Mod[] mods = ModManager.Instance.GetAllMods(true);
for (int i = mods.Length; i-- > 0;)
{
if (mods[i].AssetBundle.Contains(modelName))
{
GameObject go = mods[i].GetAsset<GameObject>(modelName, true);
if (go != null)
{
InstantiateCustomFlat(go, ref position, parent, archive, record, inDungeon);
return go;
}
Debug.LogError("Failed to import " + modelName + " from " + mods[i].Title + " as GameObject.");
}
}
return null;
}
/// <summary>
/// Check existence of gameobject for billboard in Resources
/// </summary>
/// <returns>Bool</returns>
static public bool ReplacementFlatExist(int archive, int record)
{
if (DaggerfallUnity.Settings.MeshAndTextureReplacement //check .ini setting
&& Resources.Load("Flats/" + archive.ToString() + "_" + record.ToString() + "/" + archive.ToString() + "_" + record.ToString()) != null)
return true;
return false;
}
/// <summary>
/// Import gameobject from Resources
/// </summary>
static public GameObject LoadReplacementFlat(int archive, int record, Vector3 position, Transform parent, bool inDungeon = false)
{
GameObject object3D = GameObject.Instantiate(Resources.Load("Flats/" + archive.ToString() + "_" + record.ToString() + "/" + archive.ToString() + "_" + record.ToString()) as GameObject);
InstantiateCustomFlat(object3D, ref position, parent, archive, record, inDungeon);
return object3D;
}
/// <summary>
/// Checks if dungeon flat should have a torch sound.
/// </summary>
static public bool HasTorchSound (int archive, int record)
{
if (archive == TextureReader.LightsTextureArchive)
{
switch (record)
{
case 0:
case 1:
case 6:
case 16:
case 17:
case 18:
case 19:
case 20:
return true;
}
}
return false;
}
#endregion
#region Private Methods
/// <summary>
/// Check if is winter and we are in a location which supports winter models.
/// </summary>
static private bool IsWinter()
{
return ((GameManager.Instance.PlayerGPS.ClimateSettings.ClimateType != DaggerfallConnect.DFLocation.ClimateBaseType.Desert)
&& (DaggerfallUnity.Instance.WorldTime.Now.SeasonValue == DaggerfallDateTime.Seasons.Winter));
}
/// <summary>
/// Try loading AssetBundle and check if it contains the GameObject.
/// </summary>
/// <param name="path">Location of the AssetBundle.</param>
/// <param name="modelName">Name of GameObject.</param>
/// <param name="assetBundle">Loaded AssetBundle.</param>
/// <returns>True if AssetBundle is loaded.</returns>
static private bool TryGetAssetBundle (string path, string modelName, out AssetBundle assetBundle)
{
var loadedAssetBundle = AssetBundle.LoadFromFile(path);
if (loadedAssetBundle != null)
{
// Check if AssetBundle contain the model we are looking for
if (loadedAssetBundle.Contains(modelName))
{
assetBundle = loadedAssetBundle;
return true;
}
else
loadedAssetBundle.Unload(false);
}
Debug.LogError("Error with AssetBundle: " + path + " doesn't contain " +
modelName + " or is corrupted.");
assetBundle = null;
return false;
}
/// <summary>
/// Assign parent, position, rotation and texture filtermode.
/// </summary>
static private void InstantiateCustomModel(GameObject go, ref Vector3 position, Transform parent, ref Quaternion rotation, string modelName)
{
// Update Position
go.transform.parent = parent;
go.transform.position = position;
go.transform.rotation = rotation;
// Finalise gameobject
FinaliseCustomGameObject(ref go, modelName);
}
/// <summary>
/// Assign parent, position, rotation and texture filtermode.
/// </summary>
static private void InstantiateCustomFlat(GameObject go, ref Vector3 position, Transform parent, int archive, int record, bool inDungeon)
{
// Update Position
if (inDungeon)
GetFixedPosition(ref position, archive, record);
go.transform.parent = parent;
go.transform.localPosition = position;
// Assign a random rotation so that flats in group won't look all aligned.
// We use a seed becuse we want the models to have the same
// rotation every time the same location is loaded.
if (go.GetComponent<FaceWall>() == null)
{
Random.InitState((int)position.x);
go.transform.Rotate(0, Random.Range(0f, 360f), 0);
}
// Finalise gameobject
FinaliseCustomGameObject(ref go, archive.ToString() + "_" + record.ToString());
}
/// <summary>
/// Assign texture filtermode as user settings and check integrity of materials.
/// Import textures from disk if available. This is for consistency when custom models
/// use one or more vanilla textures and the user has installed a texture pack.
/// Vanilla textures should follow the nomenclature (archive_record-frame.png) while unique
/// textures should have unique names (MyModelPack_WoodChair2.png) to avoid involuntary replacements.
/// This can also be used to import optional normal maps.
/// </summary>
/// <param name="object3D">Custom prefab</param>
/// <param name="ModelName">ID of model or sprite to be replaced. Used for debugging</param>
static private void FinaliseCustomGameObject(ref GameObject object3D, string ModelName)
{
// Get MeshRenderer
MeshRenderer meshRenderer = object3D.GetComponent<MeshRenderer>();
// Check all materials
for (int i = 0; i < meshRenderer.materials.Length; i++)
{
if (meshRenderer.materials[i].mainTexture != null)
{
// Get name of texture
string textureName = meshRenderer.materials[i].mainTexture.name;
// Use texture(s) from disk if available
// Albedo
if (TextureReplacement.CustomTextureExist(textureName))
meshRenderer.materials[i].mainTexture = TextureReplacement.LoadCustomTexture(textureName);
// Normal map
if (TextureReplacement.CustomNormalExist(textureName))
{
meshRenderer.materials[i].EnableKeyword("_NORMALMAP"); // Enable normal map in the shader if the original material doesn't have one
meshRenderer.materials[i].SetTexture("_BumpMap", TextureReplacement.LoadCustomNormal(textureName));
}
// Emission map
if (TextureReplacement.CustomEmissionExist(textureName))
{
meshRenderer.materials[i].EnableKeyword("_EMISSION"); // Enable emission map in the shader if the original material doesn't have one
meshRenderer.materials[i].SetTexture("_EmissionMap", TextureReplacement.LoadCustomEmission(textureName));
}
// MetallicGloss map
if (TextureReplacement.CustomMetallicGlossExist(textureName))
{
meshRenderer.materials[i].EnableKeyword("_METALLICGLOSSMAP"); // Enable metallicgloss map in the shader if the original material doesn't have one
meshRenderer.materials[i].SetTexture("_MetallicGlossMap", TextureReplacement.LoadCustomMetallicGloss(textureName));
}
// Assign filtermode
meshRenderer.materials[i].mainTexture.filterMode = (FilterMode)DaggerfallUnity.Settings.MainFilterMode;
if (meshRenderer.materials[i].GetTexture("_BumpMap") != null)
meshRenderer.materials[i].GetTexture("_BumpMap").filterMode = (FilterMode)DaggerfallUnity.Settings.MainFilterMode;
if (meshRenderer.materials[i].GetTexture("_EmissionMap") != null)
meshRenderer.materials[i].GetTexture("_EmissionMap").filterMode = (FilterMode)DaggerfallUnity.Settings.MainFilterMode;
if (meshRenderer.materials[i].GetTexture("_MetallicGlossMap") != null)
meshRenderer.materials[i].GetTexture("_MetallicGlossMap").filterMode = (FilterMode)DaggerfallUnity.Settings.MainFilterMode;
}
else
Debug.LogError("Custom model " + ModelName + " is missing a material or a texture");
}
}
/// <summary>
/// Fix position of dungeon gameobjects.
/// </summary>
/// <param name="position">localPosition</param>
/// <param name="archive">Archive of billboard texture</param>
/// <param name="record">Record of billboard texture</param>
static private void GetFixedPosition (ref Vector3 position, int archive, int record)
{
// Get height
int height = ImageReader.GetImageData("TEXTURE." + archive, record, createTexture:false).height;
// Correct transform
position.y -= height / 2 * MeshReader.GlobalScale;
}
#endregion
#region Legacy Methods
// This was used to import mesh and materials separately from Resources.
// It might be useful again in the future.
//
//static public Mesh LoadReplacementModel(uint modelID, ref CachedMaterial[] cachedMaterialsOut)
//{
// // Import mesh
// GameObject object3D = Resources.Load("Models/" + modelID.ToString() + "/" + modelID.ToString() + "_mesh") as GameObject;
//
// // Import materials
// cachedMaterialsOut = new CachedMaterial[object3D.GetComponent<MeshRenderer>().sharedMaterials.Length];
//
// string materialPath;
//
// // If it's not winter or the model doesn't have a winter version, it loads default materials
// if ((DaggerfallUnity.Instance.WorldTime.Now.SeasonValue != DaggerfallDateTime.Seasons.Winter) || (Resources.Load("Models/" + modelID.ToString() + "/material_w_0") == null))
// materialPath = "/material_";
// // If it's winter and the model has a winter version, it loads winter materials
// else
// materialPath = "/material_w_";
//
// for (int i = 0; i < cachedMaterialsOut.Length; i++)
// {
// if (Resources.Load("Models/" + modelID.ToString() + materialPath + i) != null)
// {
// cachedMaterialsOut[i].material = Resources.Load("Models/" + modelID.ToString() + materialPath + i) as Material;
// if (cachedMaterialsOut[i].material.mainTexture != null)
// cachedMaterialsOut[i].material.mainTexture.filterMode = (FilterMode)DaggerfallUnity.Settings.MainFilterMode; //assign texture filtermode as user settings
// else
// Debug.LogError("Custom model " + modelID + " is missing a texture");
// }
// else
// Debug.LogError("Custom model " + modelID + " is missing a material");
// }
//
// return object3D.GetComponent<MeshFilter>().sharedMesh;
//}
//
#endregion
}
}<file_sep>// Project: Daggerfall Tools For Unity
// Copyright: Copyright (C) 2009-2016 Daggerfall Workshop
// Web Site: http://www.dfworkshop.net
// License: MIT License (http://www.opensource.org/licenses/mit-license.php)
// Source Code: https://github.com/Interkarma/daggerfall-unity
// Original Author: TheLacus
// Contributors:
//
// Notes:
//
/*
* TODO:
* 1. StreamingWorld billboards
* 2. PaperDoll CharacterLayer textures works only if resolution is the same as vanilla
* (http://forums.dfworkshop.net/viewtopic.php?f=22&p=3547&sid=6a99dbcffad1a15b08dd5e157274b772#p3547)
* 3. Terrain textures
*/
using System.IO;
using System.Collections.Generic;
using UnityEngine;
using DaggerfallWorkshop.Game.UserInterface;
namespace DaggerfallWorkshop.Utility.AssetInjection
{
/// <summary>
/// Handles import and injection of custom textures and images
/// with the purpose of providing modding support.
/// </summary>
static public class TextureReplacement
{
#region Fields & Structs
// Paths
static public string texturesPath = Path.Combine(Application.streamingAssetsPath, "Textures");
static public string imgPath = Path.Combine(texturesPath, "img");
static public string cifPath = Path.Combine(texturesPath, "cif");
/// <summary>
/// Common tags for textures maps.
/// </summary>
public struct MapTags
{
public const string Normal = "_Normal";
public const string Emission = "_Emission";
public const string MetallicGloss = "_MetallicGloss";
public const string Heightmap = "_Heightmap"; //unused
public const string Occlusion = "_Occlusion"; //unused
}
/// <summary>
/// Material components and settings for custom billboards.
/// </summary>
public struct CustomBillboard
{
public List<Texture2D> MainTexture; // List of custom albedo maps
public List<Texture2D> EmissionMap; // List of custom emission maps
public bool isEmissive; // True if billboard is emissive
public int NumberOfFrames; // number of frame textures avilable on disk
}
/// <summary>
/// Custom textures for enemies.
/// </summary>
public struct CustomEnemyMaterial
{
public bool isCustom; // True if enemy uses custom textures
public List<List<Texture2D>> MainTexture; // Textures
}
#endregion
#region Textures Import
/// <summary>
/// Search for image files on disk to use as textures on models or billboards
/// (archive_record-frame.png, for example '86_3-0.png').
/// </summary>
/// <param name="archive">Archive index from TEXTURE.XXX</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index. It's different than zero only for animated billboards.</param>
/// <returns>True if texture exists.</returns>
static public bool CustomTextureExist(int archive, int record, int frame = 0)
{
return TextureFileExist(texturesPath, archive.ToString() + "_" + record.ToString() + "-" + frame.ToString());
}
/// <summary>
/// Search for image files on disk to use as textures on models or billboards
/// (name.png).
/// </summary>
/// <param name="name">Name of texture without extension.</param>
/// <returns>True if texture exists.</returns>
static public bool CustomTextureExist(string name)
{
return TextureFileExist(texturesPath, name);
}
/// <summary>
/// Import image from disk as texture2D
/// (archive_record-frame.png, for example '86_3-0.png').
/// </summary>
/// <param name="archive">Archive index from TEXTURE.XXX</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index. It's different than zero only for animated billboards</param>
/// <returns>Texture.</returns>
static public Texture2D LoadCustomTexture(int archive, int record, int frame)
{
return ImportTextureFile(texturesPath, archive.ToString() + "_" + record.ToString() + "-" + frame.ToString());
}
/// <summary>
/// Import image from disk as texture2D
/// (name.png).
/// </summary>
/// <param name="name">Name of texture without extension.</param>
/// <returns>Texture.</returns>
static public Texture2D LoadCustomTexture(string name)
{
return ImportTextureFile(texturesPath, name);
}
/// <summary>
/// Search for image file on disk to replace .IMGs.
/// (imagefile.png, for example 'REST02I0.IMG.png').
/// </summary>
/// <param name="filename">Name of image.</param>
/// <returns>True if texture exists.</returns>
static public bool CustomImageExist(string filename)
{
return TextureFileExist(imgPath, filename);
}
/// <summary>
/// Import image from disk as texture2D
/// (imagefile.png, for example 'REST02I0.IMG.png').
/// </summary>
/// <param name="filename">Name of image.</param>
/// <returns>Image.</returns>
static public Texture2D LoadCustomImage(string filename)
{
return ImportTextureFile(imgPath, filename);
}
/// <summary>
/// Search for image file on disk to replace .CIFs and .RCIs.
/// (filename_record-frame.png, for example 'INVE16I0.CIF_1-0.png').
/// </summary>
/// <param name="filename">Name of image.</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index. It's different than zero only for weapon animations (WEAPONXX.CIF).</param>
/// <returns>True if image exists.</returns>
static public bool CustomCifExist(string filename, int record, int frame = 0)
{
return TextureFileExist(cifPath, filename + "_" + record.ToString() + "-" + frame.ToString());
}
/// <summary>
/// Import image as Texture2D to replace .CIFs and .RCIs.
/// (filename_record-frame.png, for example 'INVE16I0.CIF_1-0.png').
/// </summary>
/// <param name="filename">Name of image.</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index. It's different than zero only for weapon animations (WEAPONXX.CIF) </param>
/// <returns>Image.</returns>
static public Texture2D LoadCustomCif(string filename, int record, int frame)
{
return ImportTextureFile(cifPath, filename + "_" + record.ToString() + "-" + frame.ToString());
}
/// <summary>
/// Search for image on disk to replace .CIFs and .RCIs. for a specific metalType
/// (filename_record-frame_metalType.png, for example 'WEAPON04.CIF_0-0_Iron.Png').
/// </summary>
/// <param name="filename">Name of image.</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index. It's different than zero only for weapon animations (WEAPONXX.CIF) </param>
/// <returns>True if generic or specific image exists.</returns>
static public bool CustomCifExist(string filename, int record, int frame, MetalTypes metalType)
{
if (metalType == MetalTypes.None)
return CustomCifExist(filename, record, frame);
return TextureFileExist(cifPath, filename + "_" + record.ToString() + "-" + frame.ToString() + "_" + metalType);
}
/// <summary>
/// Import image from disk to replace .CIFs and .RCIs. for a specific metalType
/// (filename_record-frame_metalType.png', for example 'WEAPON04.CIF_0-0_Iron.Png').
/// </summary>
/// <param name="filename">Name of image.</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index. It's different than zero only for weapon animations (WEAPONXX.CIF) </param>
/// <returns>Image for this metalType or generic image if metalType is None.</returns>
static public Texture2D LoadCustomCif(string filename, int record, int frame, MetalTypes metalType)
{
if (metalType == MetalTypes.None)
return LoadCustomCif(filename, record, frame);
return ImportTextureFile(cifPath, filename + "_" + record.ToString() + "-" + frame.ToString() + "_" + metalType);
}
/// <summary>
/// Search for image file on disk to use as normal map
/// (archive_record-frame_Normal.png, for example '112_3-0_Normal.png').
/// </summary>
/// <param name="archive">Archive index from TEXTURE.XXX</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index.</param>
/// <returns>True if normal map exists.</returns>
static public bool CustomNormalExist(int archive, int record, int frame)
{
return TextureFileExist(texturesPath, archive.ToString() + "_" + record.ToString() + "-" + frame.ToString() + MapTags.Normal);
}
/// <summary>
/// Search for image file on disk to use as normal map
/// (name_Normal.png).
/// </summary>
/// <param name="name">Name of texture.</param>
/// <returns>True if normal map exists.</returns>
static public bool CustomNormalExist(string name)
{
return TextureFileExist(texturesPath, name + MapTags.Normal);
}
/// <summary>
/// Import image file from disk to use as normal map.
/// (archive_record-frame_Normal.png, for example '112_3-0_Normal.png').
/// </summary>
/// <param name="archive">Archive index from TEXTURE.XXX</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index.</param>
/// <returns>Normal map.</returns>
static public Texture2D LoadCustomNormal(int archive, int record, int frame)
{
return ImportNormalMap(texturesPath, archive.ToString() + "_" + record.ToString() + "-" + frame.ToString());
}
/// <summary>
/// Import image file from disk to use as normal map
/// (name_Normal.png).
/// </summary>
/// <param name="name">Name of texture.</param>
/// <returns>Normal map.</returns>
static public Texture2D LoadCustomNormal(string name)
{
return ImportNormalMap(texturesPath, name);
}
/// <summary>
/// Search for image file on disk to use as emission map
/// (archive_record-frame_Emission.png, for example '112_3-0_Emission.png).
/// </summary>
/// <param name="archive">Archive index from TEXTURE.XXX</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index. It's different than zero only for animated billboards.</param>
/// <returns>True if emission map exists.</returns>
static public bool CustomEmissionExist(int archive, int record, int frame)
{
return TextureFileExist(texturesPath, archive.ToString() + "_" + record.ToString() + "-" + frame.ToString() + MapTags.Emission);
}
/// <summary>
/// Search for image file on disk to use as emission map
/// (name_Emission.png)
/// </summary>
/// <param name="name">Name of texture.</param>
/// <returns>True if emission map exists.</returns>
static public bool CustomEmissionExist(string name)
{
return TextureFileExist(texturesPath, name + MapTags.Emission);
}
/// <summary>
/// Import image file from disk to use as emission map
/// (archive_record-frame_Emission.png, for example '112_3-0_Emission.png').
/// </summary>
/// <param name="archive">Archive index from TEXTURE.XXX</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index. It's different than zero only for animated billboards</param>
/// <returns>Emission map.</returns>
static public Texture2D LoadCustomEmission(int archive, int record, int frame)
{
return ImportTextureFile(texturesPath, archive.ToString() + "_" + record.ToString() + "-" + frame.ToString() + MapTags.Emission);
}
/// <summary>
/// Import image file from disk to use as emission map
/// (name_Emission.png)
/// </summary>
/// <param name="name">Name of texture.</param>
/// <returns>Emission map.</returns>
static public Texture2D LoadCustomEmission(string name)
{
return ImportTextureFile(texturesPath, name + MapTags.Emission);
}
/// <summary>
/// Search for image file on disk to use as metallic map
/// (archive_record-frame_MetallicGloss.png).
/// </summary>
/// <param name="archive">Archive index from TEXTURE.XXX</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index.</param>
/// <returns>True if MetallicGloss map exist.</returns>
static public bool CustomMetallicGlossExist(int archive, int record, int frame)
{
return TextureFileExist(texturesPath, archive.ToString() + "_" + record.ToString() + "-" + frame.ToString() + MapTags.MetallicGloss);
}
/// <summary>
/// Search for image file on disk to use as metallic map
/// (name_MetallicGloss.png).
/// </summary>
/// <param name="name">Name of texture.</param>
/// <returns>True if MetallicGloss map exist.</returns>
static public bool CustomMetallicGlossExist(string name)
{
return TextureFileExist(texturesPath, name + MapTags.MetallicGloss);
}
/// <summary>
/// Import image file from disk to use as metallic map.
/// (archive_record-frame_MetallicGloss.png).
/// </summary>
/// <param name="archive">Archive index from TEXTURE.XXX</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index.</param>
/// <returns>MetallicGloss map.</returns>
static public Texture2D LoadCustomMetallicGloss(int archive, int record, int frame)
{
return ImportTextureFile(texturesPath, archive.ToString() + "_" + record.ToString() + "-" + frame.ToString() + MapTags.MetallicGloss);
}
/// <summary>
/// Import image file from disk to use as MetallicGloss map
/// (name_MetallicGloss.png).
/// </summary>
/// <param name="name">Name of texture.</param>
/// <returns>MetallicGloss map.</returns>
static public Texture2D LoadCustomMetallicGloss(string name)
{
return ImportTextureFile(texturesPath, name + MapTags.MetallicGloss);
}
// General import methods for mods or other external code.
/// <summary>
/// Import png file from disk as Texture2D.
/// </summary>
/// <param name="path">Path where image file is located.</param>
/// <param name="name">Name of image file without extension.</param>
static public Texture2D ImportTextureFromDisk(string path, string name)
{
return ImportTextureFile(path, name);
}
/// <summary>
/// Import png file from disk as Texture2D.
/// </summary>
/// <param name="path">Path where image file is located.</param>
/// <param name="name">Name of image file without extension.</param>
/// <param name="mapTag">Tag for texture map.</param>
static public Texture2D ImportTextureFromDisk (string path, string name, string mapTag)
{
if (mapTag == MapTags.Normal)
return ImportNormalMap(path, name);
else
return ImportTextureFile(path, name + mapTag);
}
#endregion
#region Texture Injection
/// <summary>
/// Import texture(s) used on models.
/// </summary>
/// <param name="archive">Archive index</param>
/// <param name="record">Record index</param>
/// <param name="frame">Texture frame</param>
/// <param name="results">Texture Results</param>
/// <param name="GenerateNormals">Will create normal map</param>
static public void LoadCustomTextureResults(int archive, int record, int frame, ref GetTextureResults results, ref bool GenerateNormals)
{
// Main texture
results.albedoMap = LoadCustomTexture(archive, record, frame);
// Normal map
if (CustomNormalExist(archive, record, frame))
{
results.normalMap = LoadCustomNormal(archive, record, frame);
GenerateNormals = true;
}
// Emission map
// windowed walls use a custom emission map or stick with vanilla
// non-window use the main texture as emission, unless a custom map is provided
if (results.isEmissive)
{
if (CustomEmissionExist(archive, record, frame)) //import emission texture
results.emissionMap = LoadCustomEmission(archive, record, frame);
else if (!results.isWindow) //reuse albedo map for basic colour emission
results.emissionMap = results.albedoMap;
}
}
/// <summary>
/// Import additional custom components of material.
/// </summary>
/// <param name="archive">Archive index</param>
/// <param name="record">Record index</param>
/// <param name="frame">Texture frame</param>
/// <param name="material">Material.</param>
static public void CustomizeMaterial(int archive, int record, int frame, Material material)
{
// MetallicGloss map
if (CustomMetallicGlossExist(archive, record, frame))
{
material.EnableKeyword("_METALLICGLOSSMAP");
material.SetTexture("_MetallicGlossMap", LoadCustomMetallicGloss(archive, record, frame));
}
// Properties
if (XMLManager.XmlFileExist(archive, record, frame))
{
string fileName = GetName(archive, record, frame);
float value;
// Metallic parameter
if (XMLManager.TryGetFloat(fileName, "metallic", out value, texturesPath))
material.SetFloat("_Metallic", value);
// Smoothness parameter
if (XMLManager.TryGetFloat(fileName, "smoothness", out value, texturesPath))
material.SetFloat("_Glossiness", value);
}
}
/// <summary>
/// Replace texture(s) on billboard gameobject.
/// This is implemented only for interior and dungeon billboards for now
/// </summary>
/// <paran name="go">Billboard gameobject.</param>
/// <param name="archive">Archive index.</param>
/// <param name="record">Record index.</param>
static public void LoadCustomBillboardTexture(GameObject go, int archive, int record)
{
// Get MeshRenderer
MeshRenderer meshRenderer = go.GetComponent<MeshRenderer>();
// UV default values
var uv = Vector2.zero;
// Customize billboard size (scale)
if (XMLManager.XmlFileExist(archive, record))
{
// Get name of file
string name = GetName(archive, record);
// Set scale
Transform transform = go.GetComponent<Transform>();
transform.localScale = XMLManager.GetScale(name, texturesPath, transform.localScale);
go.GetComponent<DaggerfallBillboard>().SetCustomSize(archive, record, transform.localScale.y);
// Get UV
uv = XMLManager.GetUv(name, texturesPath, uv.x, uv.y);
}
// Update UV map
MeshFilter meshFilter = go.GetComponent<MeshFilter>();
UpdateUV(meshFilter, uv.x, uv.y);
// Check if billboard is emissive
bool isEmissive = false;
if (meshRenderer.materials[0].GetTexture("_EmissionMap") != null)
isEmissive = true;
// Import texture(s)
Texture2D albedoTexture, emissionMap;
LoadCustomBillboardFrameTexture(isEmissive, out albedoTexture, out emissionMap, archive, record);
// Main texture
meshRenderer.materials[0].SetTexture("_MainTex", albedoTexture);
// Emission maps for lights
if (isEmissive)
meshRenderer.materials[0].SetTexture("_EmissionMap", emissionMap);
// Check if billboard is animated
int NumberOfFrames = NumberOfAvailableFrames(archive, record);
if (NumberOfFrames > 1)
{
// Import textures for each frame
go.GetComponent<DaggerfallBillboard>().SetCustomMaterial(archive, record, NumberOfFrames, isEmissive);
}
}
static public void SetupCustomEnemyMaterial(ref MeshRenderer meshRenderer, ref MeshFilter meshFilter, int archive)
{
// Set Main Texture
Texture2D albedoTexture = LoadCustomTexture(archive, 0, 0);
albedoTexture.filterMode = (FilterMode)DaggerfallUnity.Settings.MainFilterMode;
meshRenderer.material.mainTexture = albedoTexture;
// Update UV map
UpdateUV(meshFilter);
}
/// <summary>
/// Import custom texture and label settings for buttons
/// </summary>
/// <param name="button">Button</param>
/// <param name="colorName">Name of texture</param>
static public void SetCustomButton(ref Button button, string colorName)
{
// Load texture
button.BackgroundTexture = LoadCustomTexture(colorName);
button.BackgroundTexture.filterMode = (FilterMode)DaggerfallUnity.Settings.GUIFilterMode;
// Load settings from Xml
if (XMLManager.XmlFileExist(colorName, texturesPath))
{
// Set custom color
if (XMLManager.GetString(colorName, "customtext", texturesPath) == "true")
button.Label.TextColor = XMLManager.GetColor(colorName, texturesPath);
// Disable text. This is useful if text is drawn on texture
else if (XMLManager.GetString(colorName, "customtext", texturesPath) == "notext")
button.Label.Text = "";
}
}
#endregion
#region Utilities
/// <summary>
/// Convert (archive, record, frame) to string name.
/// </summary>
/// <param name="archive">Archive index from TEXTURE.XXX</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index. It's different than zero only for animations.</param>
static public string GetName (int archive, int record, int frame = 0)
{
return archive.ToString() + "_" + record.ToString() + "-" + frame.ToString();
}
/// <summary>
/// Convert (filename, record, frame) to string name.
/// </summary>
/// <param name="filename">Name of CIF/RCI file.</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index. It's different than zero only for animations.</param>
static public string GetNameCifRci (string filename, int record, int frame = 0)
{
return filename + "_" + record.ToString() + "-" + frame.ToString();
}
/// <summary>
/// Import texture(s) for billboard gameobject for specified frame.
/// </summary>
/// <paran name="isEmissive">True for lights.</param>
/// <paran name="albedoTexture">Main texture for this frame.</param>
/// <paran name="emissionMap">Eventual Emission map for this frame.</param>
/// <param name="archive">Archive index.</param>
/// <param name="record">Record index.</param>
/// <param name="frame">Frame index. It's different than zero only for animated billboards.</param>
static public void LoadCustomBillboardFrameTexture(bool isEmissive, out Texture2D albedoTexture, out Texture2D emissionMap, int archive, int record, int frame = 0)
{
// Main texture
albedoTexture = LoadCustomTexture(archive, record, frame);
albedoTexture.filterMode = (FilterMode)DaggerfallUnity.Settings.MainFilterMode;
// Emission map
if (isEmissive)
{
// Import emission map if available on disk
if (CustomEmissionExist(archive, record, frame))
emissionMap = LoadCustomEmission(archive, record, frame);
// If texture is emissive but no emission map is provided, emits from the whole surface
else
emissionMap = albedoTexture;
emissionMap.filterMode = (FilterMode)DaggerfallUnity.Settings.MainFilterMode;
}
else
emissionMap = null;
}
/// <summary>
/// Get size of vanilla texture, even if using a custom one.
/// </summary>
/// <param name="texture">Texture.</param>
/// <param name="textureName">Archive of texture.</param>
/// <param name="record">Record of texture.</param>
/// <param name="frame">Frame of texture.</param>
/// <returns>Vector2 with width and height</returns>
static public Vector2 GetSizeFromTexture(Texture2D texture, int archive, int record = 0, int frame = 0)
{
if (CustomTextureExist(archive, record, frame))
{
ImageData imageData = ImageReader.GetImageData("TEXTURE." + archive, createTexture: false);
return new Vector2(imageData.width, imageData.height);
}
else
return new Vector2(texture.width, texture.height);
}
/// <summary>
/// Get size of vanilla texture, even if using a custom one.
/// </summary>
/// <param name="texture">Texture.</param>
/// <param name="textureName">Name of texture file.</param>
/// <param name="record">Record of texture (CifRci only).</param>
/// <param name="frame">Frame of texture (CifRci only).</param>
/// <returns>Vector2 with width and height</returns>
static public Vector2 GetSizeFromTexture(Texture2D texture, string textureName, int record = 0, int frame = 0)
{
if (CustomImageExist(textureName) || CustomCifExist(textureName, record, frame))
{
ImageData imageData = ImageReader.GetImageData(textureName, createTexture: false);
return new Vector2(imageData.width, imageData.height);
}
else
return new Vector2(texture.width, texture.height);
}
#endregion
#region Private Methods
/// <summary>
/// Check if image file exist on disk.
/// </summary>
/// <param name="path">Location of image file.</param>
/// <param name="name">Name of image file.</param>
/// <returns></returns>
static private bool TextureFileExist (string path, string name)
{
if (DaggerfallUnity.Settings.MeshAndTextureReplacement //check .ini setting
&& File.Exists(Path.Combine(path, name + ".png")))
return true;
return false;
}
/// <summary>
/// Import image file with .png extension from disk,
/// to be used as a texture.
/// </summary>
/// <param name="path">Location of image file.</param>
/// <param name="name">Name of image file.</param>
/// <returns></returns>
static private Texture2D ImportTextureFile (string path, string name)
{
// Create empty texture, size will be the actual size of .png file
Texture2D tex = new Texture2D(2, 2);
// Load image as Texture2D
tex.LoadImage(File.ReadAllBytes(Path.Combine(path, name + ".png")));
// Return imported texture
if (tex != null)
return tex;
Debug.LogError("Can't import custom texture " + name + ".png from " + path);
return null;
}
/// <summary>
/// Import image file with .png extension from disk,
/// to be used as a normal map.
/// </summary>
/// <param name="path">Location of image file.</param>
/// <param name="name">Name of image file.</param>
/// <returns>Normal map as Texture2D</returns>
static private Texture2D ImportNormalMap (string path, string name)
{
//create empty texture, size will be the actual size of .png file
Texture2D tex = new Texture2D(2, 2, TextureFormat.ARGB32, true);
// Load image as Texture2D
tex.LoadImage(File.ReadAllBytes(Path.Combine(path, name + MapTags.Normal + ".png")));
if (tex == null)
{
Debug.LogError("Can't import custom texture " + name + ".png from " + path);
return null;
}
Color32[] colours = tex.GetPixels32();
for (int i = 0; i < colours.Length; i++)
{
colours[i].a = colours[i].r;
colours[i].r = colours[i].b = colours[i].g;
}
tex.SetPixels32(colours);
tex.Apply();
return tex;
}
/// <summary>
/// Update UV map
/// </summary>
/// <param name="meshFilter">MeshFilter of GameObject</param>
static private void UpdateUV (MeshFilter meshFilter, float x = 0, float y = 0)
{
Vector2[] uv = new Vector2[4];
uv[0] = new Vector2(x, 1 - y);
uv[1] = new Vector2(1 - x, 1 - y);
uv[2] = new Vector2(x, y);
uv[3] = new Vector2(1 - x, y);
meshFilter.mesh.uv = uv;
}
/// <summary>
/// Check all frames available on disk.
/// </summary>
/// <param name="archive">Archive index.</param>
/// <param name="record">Record index.</param>
/// <returns>Number of textures present on disk for this record</returns>
static private int NumberOfAvailableFrames(int archive, int record)
{
int frames = 0;
while (CustomTextureExist(archive, record, frames))
{
frames++;
}
return frames;
}
#endregion
}
}
| 3f4988571f4c52649e985189520f000ebde2cb69 | [
"C#"
] | 3 | C# | midopa/daggerfall-unity | 5e98cf9190cfda4e8050dd76233565b66be3f598 | a1bff2294c481516a446e559aabe0c338882cc10 |
refs/heads/master | <file_sep>extern crate anyhow;
extern crate async_std;
extern crate log;
extern crate pest;
extern crate pest_derive;
extern crate pretty_env_logger;
extern crate serde;
extern crate serde_json;
extern crate surf;
mod parser;
mod update;
use anyhow::Error;
use async_std::fs;
use clap::Clap;
use log::{debug, info};
use parser::NSUpdateQueue;
use update::RequestQueue;
use std::env;
use std::panic;
// Constants
const PKG_LOG_LEVEL_VAR: &str = "NSUPDATE_CLOUDFLARE_LOG";
const PKG_LOG_LEVEL_DEFAULT: &str = "nsupdate_cloudflare=info";
const PKG_LOG_LEVEL_VERBOSE_1: &str = "info";
const PKG_LOG_LEVEL_VERBOSE_2: &str = "debug";
const PKG_VERSION: &str = env!("CARGO_PKG_VERSION");
const PKG_DESCRIPTION: &str = env!("CARGO_PKG_DESCRIPTION");
#[derive(Clap)]
#[clap(version = PKG_VERSION, about = PKG_DESCRIPTION)]
struct Opts {
#[clap(short = "f", long = "file", about = "Path to nsupdate file")]
file: String,
#[clap(
short = "z",
long = "zone",
about = "Zone ID retrieved from Cloudflare"
)]
zone_id: String,
#[clap(short = "t", long = "token", about = "Token retrieved from Cloudflare")]
token: String,
#[clap(
short = "v",
long = "verbose",
about = "Verbose level",
parse(from_occurrences)
)]
verbose: i8,
}
async fn execute(input: String, zone_id: &str, token: &str) -> Result<(), Error> {
let mut input_text = Some(input);
let mut batch_count: usize = 0;
let mut total: usize = 0;
let mut total_failed: usize = 0;
while input_text.is_some() {
// Well, too much hassle for using recursion in async fn
batch_count += 1;
let mut parse_result = NSUpdateQueue::new().await;
let remaining = parse_result.parse_text(&input_text.expect("Wut?")).await?;
info!(
"{} commands in batch {}",
parse_result.len().await,
batch_count
);
debug!("Parse result: {:?}", &parse_result);
if parse_result.has_send().await {
let request_queue = RequestQueue::from(parse_result);
let (subtotal, subtotal_failed) = request_queue.process(zone_id, token).await?;
total += subtotal;
total_failed += total_failed;
info!(
"Batch {} Subtotal: Processed {} requests, {} failed",
batch_count, subtotal, subtotal_failed
);
} else {
info!("No \"send\" command found, nothing to do...");
}
input_text = remaining;
}
info!(
"Processed {} requests in total, {} failed",
total, total_failed
);
Ok(())
}
/// Set panic hook with repository information
fn setup_panic_hook() {
panic::set_hook(Box::new(|panic_info: &panic::PanicInfo| {
if let Some(info) = panic_info.payload().downcast_ref::<&str>() {
println!("Panic occurred: {:?}", info);
} else {
println!("Panic occurred");
}
if let Some(location) = panic_info.location() {
println!(
r#"In file "{}" at line "{}""#,
location.file(),
location.line()
);
}
println!(
"Please report this panic to {}/issues",
env!("CARGO_PKG_REPOSITORY")
);
}));
}
#[async_std::main]
async fn main() -> Result<(), Error> {
// Setup panic hook
setup_panic_hook();
// Parse command line options
let opts: Opts = Opts::parse();
// Setup logger
if env::var(PKG_LOG_LEVEL_VAR).is_err() {
match opts.verbose {
0 => env::set_var(PKG_LOG_LEVEL_VAR, PKG_LOG_LEVEL_DEFAULT),
1 => env::set_var(PKG_LOG_LEVEL_VAR, PKG_LOG_LEVEL_VERBOSE_1),
2 | _ => env::set_var(PKG_LOG_LEVEL_VAR, PKG_LOG_LEVEL_VERBOSE_2),
}
}
pretty_env_logger::try_init_custom_env(PKG_LOG_LEVEL_VAR)?;
info!("Reading nsupdate file...");
let unparsed_file = fs::read_to_string(opts.file).await?;
info!("Start parsing...");
execute(unparsed_file, &opts.zone_id, &opts.token).await
}
<file_sep>[package]
name = "nsupdate_cloudflare"
version = "0.1.0"
authors = [ "<NAME> <<EMAIL>>" ]
edition = "2018"
description = "A simple yet dirty tool to apply nsupdate commands to CloudFlare DNS"
homepage = "https://github.com/RedL0tus/nsupdate_cloudflare"
repository = "https://github.com/RedL0tus/nsupdate_cloudflare"
keywords = [ "nsupdate", "cloudflare", "dns" ]
readme = "README.md"
license = "MIT"
categories = [ "command-line-utilities" ]
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html
[dependencies]
log = "^0.4"
clap = "3.0.0-beta.1"
pest = "^2.1"
surf = "2.0.0-alpha.2"
serde = { version = "^1.0", features = [ "derive" ] }
anyhow = "^1.0"
async-std = { version = "^1.5", features = [ "attributes" ] }
serde_json = "^1.0"
pest_derive = "^2.1"
pretty_env_logger = "^0.4"
<file_sep>use anyhow::{bail, Error};
use log::{debug, info, warn};
use serde::{Deserialize, Serialize};
use serde_json::json;
use super::parser::NSUpdateAction;
use super::parser::NSUpdateActionAdd;
use super::parser::NSUpdateActionDelete;
use super::parser::NSUpdateCommand;
use super::parser::NSUpdateQueue;
#[derive(Debug, Serialize)]
pub struct RequestDataAdd {
#[serde(rename = "type")]
record_type: String,
name: String,
content: String,
ttl: usize,
priority: Option<usize>,
proxied: bool,
}
#[derive(Clone, Debug)]
pub struct RequestDataDelete {
pub record_type: String,
pub name: String,
}
#[derive(Debug)]
pub struct RequestQueue {
inner: Vec<RequestData>,
}
#[derive(Clone, Debug, Default, Deserialize)]
struct CFRecord {
id: String,
#[serde(default)]
#[serde(rename = "type")]
record_type: String,
#[serde(default)]
name: String,
#[serde(default)]
content: String,
#[serde(default)]
ttl: usize,
#[serde(default)]
locked: bool,
#[serde(default)]
zone_id: String,
#[serde(default)]
zone_name: String,
}
#[derive(Clone, Debug, Default, Deserialize)]
struct CFError {
code: usize,
message: String,
}
#[derive(Debug, Default, Deserialize)]
struct CFResultInfo {
page: usize,
per_page: usize,
count: usize,
total_count: usize,
pub total_pages: usize,
}
#[derive(Debug, Default, Deserialize)]
struct CFListResponse {
success: bool,
errors: Vec<CFError>,
#[serde(skip)]
messages: (),
result: Vec<CFRecord>,
result_info: CFResultInfo,
}
#[derive(Debug, Default)]
struct CFCurrentRecords {
inner: Vec<CFRecord>,
}
#[derive(Clone, Debug, Deserialize)]
struct CFUpdateResponse {
success: bool,
errors: Vec<CFError>,
result: Option<CFRecord>,
}
#[derive(Debug)]
pub enum RequestData {
Add(RequestDataAdd),
Delete(RequestDataDelete),
}
impl From<NSUpdateActionAdd> for RequestDataAdd {
fn from(source: NSUpdateActionAdd) -> Self {
Self {
record_type: source.record_type,
name: source.domain,
content: source.content,
ttl: source.ttl,
priority: source.priority,
proxied: false,
}
}
}
impl From<NSUpdateActionDelete> for RequestDataDelete {
fn from(source: NSUpdateActionDelete) -> Self {
Self {
record_type: source.record_type,
name: source.domain,
}
}
}
impl From<NSUpdateQueue> for RequestQueue {
fn from(source: NSUpdateQueue) -> Self {
Self {
inner: source
.into_inner()
.into_iter()
.filter_map(|command| {
if let NSUpdateCommand::Update(update) = command {
Some(match update {
NSUpdateAction::Add(orig_add) => {
RequestData::Add(RequestDataAdd::from(orig_add))
}
NSUpdateAction::Delete(orig_delete) => {
RequestData::Delete(RequestDataDelete::from(orig_delete))
}
})
} else {
None
}
})
.collect(),
}
}
}
impl CFListResponse {
async fn new(zone_id: &str, token: &str, page: usize) -> Result<Self, Error> {
match surf::get(format!(
"https://api.cloudflare.com/client/v4/zones/{}/dns_records?per_page=1000&page={}",
zone_id, page
))
.set_header(
"Authorization".parse().expect("Wut?"),
format!("Bearer {}", token),
)
.recv_json()
.await
{
// Surf sucks at this part
Ok(response) => Ok(response),
Err(err) => bail!(err),
}
}
async fn get_records(self) -> Result<Vec<CFRecord>, Error> {
Ok(self.result)
}
async fn get_total_pages(&self) -> Result<usize, Error> {
if !self.success {
bail!(format!(
"Failed to request records from CloudFlare: {:?}",
&self.errors
));
}
Ok(self.result_info.total_pages)
}
}
impl CFCurrentRecords {
async fn new() -> Self {
Self {
..Default::default()
}
}
async fn append(&mut self, result: &mut Vec<CFRecord>) {
debug!(">>> Appending : {:?}", &result);
self.inner.append(result);
}
async fn update(&mut self, zone_id: &str, token: &str) -> Result<(), Error> {
info!("Updating record list");
let first_record = CFListResponse::new(zone_id, token, 1).await?;
let total_pages = first_record.get_total_pages().await?;
self.append(&mut first_record.get_records().await?).await;
if total_pages > 1 {
for page in 2usize..=total_pages {
self.append(
&mut CFListResponse::new(zone_id, token, page)
.await?
.get_records()
.await?,
)
.await;
}
}
info!("Received {} records", self.inner.len());
debug!("Current records: {:?}", &self);
Ok(())
}
async fn find_record(&self, name: &str, record_type: &str) -> Result<Option<&CFRecord>, Error> {
if self.inner.is_empty() {
Ok(None)
} else {
debug!("Finding record ID");
Ok(self.inner.iter().find(|&record| {
debug!(
">>> Target: {:?}, current: {:?}",
format!("{}.", &record.name),
name
);
(format!("{}.", record.name) == name) && (record.record_type == record_type)
}))
}
}
async fn find_record_id(&self, name: &str, record_type: &str) -> Result<Option<&str>, Error> {
if let Some(record) = self.find_record(name, record_type).await? {
Ok(Some(&record.id))
} else {
Ok(None)
}
}
}
impl RequestDataAdd {
async fn send(self, zone_id: &str, token: &str) -> Result<Option<CFUpdateResponse>, Error> {
info!("Adding {}", self.name);
match surf::post(format!(
"https://api.cloudflare.com/client/v4/zones/{}/dns_records",
zone_id
))
.set_header(
"Authorization".parse().expect("Wut?"),
format!("Bearer {}", token),
)
.body_json(&json!(self))?
.recv_json()
.await
{
Ok(response) => Ok(Some(response)),
Err(err) => bail!(err),
}
}
}
impl RequestDataDelete {
async fn send(
self,
zone_id: &str,
token: &str,
record_id: Option<&str>,
) -> Result<Option<CFUpdateResponse>, Error> {
info!("Deleting {}", self.name);
if record_id.is_none() {
warn!("Record not found");
return Ok(None);
}
match surf::delete(format!(
"https://api.cloudflare.com/client/v4/zones/{}/dns_records/{}",
zone_id,
record_id.expect("Wut?")
))
.set_header(
"Authorization".parse().expect("Wut?"),
format!("Bearer {}", token),
)
.recv_json()
.await
{
Ok(response) => Ok(Some(response)),
Err(err) => bail!(err),
}
}
}
impl RequestData {
async fn send(
self,
zone_id: &str,
token: &str,
current_records: &CFCurrentRecords,
) -> Result<Option<CFUpdateResponse>, Error> {
Ok(match self {
Self::Add(request_add) => request_add.send(zone_id, token).await?,
Self::Delete(request_delete) => {
let domain = request_delete.clone().name;
let record_type = request_delete.clone().record_type;
let record_id = current_records
.find_record_id(&domain, &record_type)
.await?;
debug!("Record ID: {:?}, domain: {:?}", &record_id, &domain);
request_delete.send(zone_id, token, record_id).await?
}
})
}
}
impl RequestQueue {
pub async fn process(self, zone_id: &str, token: &str) -> Result<(usize, usize), Error> {
let mut current_records = CFCurrentRecords::new().await;
current_records.update(zone_id, token).await?;
let iterator = self.inner.into_iter();
let mut subtotal: usize = 0;
let mut subtotal_failed: usize = 0;
for request in iterator {
let result = request.send(zone_id, token, ¤t_records).await?;
subtotal += 1;
info!("Result: {}", {
if result.is_some() && result.clone().expect("Wut?").success {
"SUCCESS"
} else {
subtotal_failed += 1;
"FAILED"
}
});
debug!("Result: {:?}", result);
}
Ok((subtotal, subtotal_failed))
}
}
<file_sep>nsupdate_cloudflare
===================
A simple yet dirty tool that can update your DNS records on CloudFlare with good old nsupdate commands.
I know the `src/parser.rs` and `src/nsupdate.pest` is dirty as fsck, but hey, it werks.
Usage
-----
```
nsupdate_cloudflare 0.1.0
A simple yet dirty tool to apply nsupdate commands to CloudFlare DNS
USAGE:
nsupdate_cloudflare [FLAGS] --file <file> --zone <zone-id> --token <token>
FLAGS:
-h, --help Prints help information
-v, --verbose Verbose level
-V, --version Prints version information
OPTIONS:
-f, --file <file> Path to nsupdate file
-t, --token <token> Token retrieved from Cloudflare
-z, --zone <zone-id> Zone ID retrieved from Cloudflare
```
<file_sep>use pest::Parser;
use pest_derive::Parser;
use anyhow::Error;
#[derive(Parser)]
#[grammar = "nsupdate.pest"]
struct NSUpdateParser;
#[derive(Debug)]
pub struct NSUpdateActionAdd {
pub domain: String,
pub ttl: usize,
pub record_type: String,
pub priority: Option<usize>,
pub content: String,
}
#[derive(Debug)]
pub struct NSUpdateActionDelete {
pub domain: String,
pub record_type: String,
}
#[derive(Debug)]
pub enum NSUpdateAction {
Add(NSUpdateActionAdd),
Delete(NSUpdateActionDelete),
}
#[derive(Debug)]
pub enum NSUpdateCommand {
Update(NSUpdateAction),
Send,
}
#[derive(Debug, Default)]
pub struct NSUpdateQueue {
inner: Vec<NSUpdateCommand>,
send: bool,
}
impl NSUpdateQueue {
pub async fn new() -> Self {
Self {
send: false,
..Default::default()
}
}
async fn push(&mut self, command: NSUpdateCommand) {
self.inner.push(command);
}
async fn set_send(&mut self) {
self.send = true;
}
pub async fn has_send(&self) -> bool {
self.send
}
pub fn into_inner(self) -> Vec<NSUpdateCommand> {
self.inner
}
// Separated because I'm going to make some kind of REPL with it
pub async fn parse_text(&mut self, input: &str) -> Result<Option<String>, Error> {
let mut lines = input.split("\n").into_iter();
while !self.has_send().await {
if let Some(line) = lines.next() {
self.parse_command(line).await?;
} else {
break;
}
}
let remaining: String = lines.collect::<Vec<&str>>().join("\n");
Ok(if remaining.len() > 0 {
Some(remaining)
} else {
None
})
}
pub async fn len(&self) -> usize {
self.inner.len()
}
// These are surely garbage code, but it just werks.
pub async fn parse_command(&mut self, input: &str) -> Result<(), Error> {
let input_pairs = NSUpdateParser::parse(Rule::line, input)?;
for command in input_pairs {
match command.as_rule() {
Rule::update => {
for action in command.into_inner() {
self.push(NSUpdateCommand::Update({
match action.as_rule() {
Rule::add => {
let mut parameters = action.into_inner();
let domain = parameters.next().unwrap().as_str().to_string();
let ttl = parameters.next().unwrap().as_str().parse()?;
let record_type = parameters
.clone()
.skip(1)
.next()
.unwrap()
.as_str()
.to_string();
let (priority, content) = if parameters
.clone()
.skip(3)
.next()
.is_none()
{
(
None,
parameters.skip(2).next().unwrap().as_str().to_string(),
)
} else {
(
Some(
parameters
.clone()
.skip(2)
.next()
.unwrap()
.as_str()
.parse()?,
),
parameters.skip(3).next().unwrap().as_str().to_string(),
)
};
NSUpdateAction::Add(NSUpdateActionAdd {
domain,
ttl,
record_type,
priority,
content,
})
}
Rule::delete => {
let mut parameters = action.into_inner();
NSUpdateAction::Delete(NSUpdateActionDelete {
domain: parameters.next().unwrap().as_str().to_string(),
record_type: parameters
.next()
.unwrap()
.as_str()
.to_string(),
})
}
_ => unreachable!(),
}
}))
.await
}
}
Rule::send => {
self.push(NSUpdateCommand::Send).await;
self.set_send().await;
}
Rule::EOI | Rule::WHITESPACE | Rule::COMMENT => continue,
_ => unreachable!(),
}
}
Ok(())
}
}
| d73f5a506a71b37fea9749c0184f8cd2d5a07945 | [
"TOML",
"Rust",
"Markdown"
] | 5 | Rust | RedL0tus/nsupdate_cloudflare | 79df37fe2163c257da5fd56606397616e6ce7fa4 | dc50f07a4bb115660045223effafb79d4d63acb0 |
refs/heads/master | <file_sep>package jkatto.cse.edu.learningfragments;
import android.support.v4.app.FragmentManager;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
public class MainActivity extends AppCompatActivity implements FirstFragment.OnFragmentInteractionListener {
//10-
FirstFragment firstFragment = null;
//-11
SecondFragment secondFragment = null;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//12-
FragmentManager fragmentManager = getSupportFragmentManager();
firstFragment = (FirstFragment) fragmentManager.findFragmentById(R.id.firstFragmnet);
secondFragment = (SecondFragment) fragmentManager.findFragmentById(R.id.SecondFragment);
}
//8-
public void setMessageText(String text){
secondFragment.setMessageText(text);
}
//9-
public void setMessageTextSize(int size){
secondFragment.setMessageTextSize(size);
}
}
| e73806e390ed507687aa9e15405a260761e16e83 | [
"Java"
] | 1 | Java | jasonkatto/LearningFragments | bedae48a59a63b5d53704e20c2898bf8f1a1f95c | c6d3eee0fa6eefca771ab509b4b64542187defcf |
refs/heads/master | <repo_name>silverstripe-scienceninjas/sapphire<file_sep>/docs/en/tutorials/1-building-a-basic-site.md
# Tutorial 1 - Building a Basic Site
## Overview
Welcome to the first in this series of tutorials on the SilverStripe Content Management System (CMS).
These tutorials are designed to take you from an absolute beginner to being able to build large, complex websites with
SilverStripe. We assume to begin with, that you have some XHTML, CSS and PHP knowledge. This first tutorial provides an absolute
introduction to building a simple website using SilverStripe. It will also teach you how to use the content management system at a basic level.
## What are we working towards?
We are going to create a site in which all the content can be edited in the SilverStripe CMS. It will have a two-level
navigation system, which will be generated on the fly to include all pages you add in the CMS. We will use two different
templates - one for the home page, and one for the rest of the site.

## Installation
You need to [download the SilverStripe software](http://www.silverstripe.org/stable-download) and install it to your local
machine or to a webserver.
For more infomation about installing and configuring a webserver read the [Installation instructions and videos](../installation).
If you want to follow this tutorial please choose "empty template" when installing SilverStripe. If you want a fully
featured theme then select the 'BlackCandy' option.
## Exploring the installation
After installation, open up the folder where you installed SilverStripe. If you installed on windows with WAMP, it will
likely be at *c:\wamp\wwww*.
Let's have a look at the folder structure.
| Directory | | Description |
| --------- | | ----------- |
| assets/ | | Contains images and other files uploaded via the SilverStripe CMS. You can also place your own content inside it, and link to it from within the content area of the CMS. |
| cms/ | | Contains all the files that form the CMS area of your site. It’s structure is similiar to the mysite/ directory, so if you find something interesting, it should be easy enough to look inside and see how it was built. |
| framework/ | | The framework that builds both your own site and as the CMS that powers it. You’ll be utilizing files in this directory often, both directly and indirectly. |
| mysite/ | | Contains all your sites code (mainly PHP and JavaScript) |
| themes/ | | Combines all images, stylesheets and templates powering your website into a reusable "theme" |
When designing your site you should only need to modify the *mysite*, *themes* and *assets* folders. The rest of the folders contain files and data that are not specific to any site.
## Using the CMS
The CMS is the area in which you can manage your site content. You can access the cms at http://localhost/admin. You
will be presented with a login screen. You can login with the details you provided at installation. After logging in you
should be greeted with the CMS, pictured below (we've entered some test content).

1. These buttons allow you to move between the different sections in the CMS. There are three core sections in the CMS - Site Content, Files & Images and Security. Modules may have their own sections here as well, if any are installed. In this tutorial we will be focusing on the Site Content section.
2. All the pages in the site are laid out in the site tree. You can add, delete and reorganize the pages using the buttons at the top. Clicking on a page will open it in the editor on the right.
3. This section allows you to edit the content for the currently selected page, as well as changing other properties of the page such as the page name and URL. The content editor has full [WYSIWYG](http://en.wikipedia.org/wiki/WYSIWYG) abilities, allow you to change formatting and insert links, images and tables.
4. There are two copies of each page: draft & published. These buttons allow you to save your changes to the draft copy, publish your draft copy, or revert your draft copy to the published copy. By having separate draft & published copies, we can preview draft changes in the site before publishing them to the live site.
5. The navigator will open the current page in the CMS, the draft site, or the published site.
### Try it
There are three pages already created for you - "Home", "About Us" and "Contact Us", as well as a 404 page. Experiment
with the editor - try different formatting, tables and images. When you are done, click "Save" to save the page or "Save
& Publish" to post the content to the live site. In our screenshot we've entered some test content.
> Don't worry that we currently have no way of navigating from page to page without using the CMS - we will build a navigation system soon.
When you create a new page, you are given a drop down that allows you to select the page type of the page. The page type
specifies the templates used to render the page, the fields that are able to be edited in the CMS, and page specific
behavior. We will explain page types in more depth as we progress; for now, make all pages of the type "Page".

**SilverStripe's virtual URLs**
While you are on the draft or live SilverStripe site, you may notice the URLs point to files that don't exist, e.g.
http://localhost/contact. SilverStripe uses the URL field on the Meta-Data tab of the editor to look up the appropriate
page in the database.

When you create a new page, SilverStripe automatically creates an appropriate URL for it. For example, *About Us* will
become *about-us*. You are able to change it yourself so that you can make long titles more usable or descriptive. For
example, *Employment Opportunities* could be shortened to *jobs*. The ability to generate easy to type, descriptive URLs
for SilverStripe pages improves accessibility for humans and search engines.
You should ensure the URL for the home page is *home*. By default, SilverStripe loads the page with the URL *home*.
## Templates
All pages on a SilverStripe site are rendered using a template. A template is an HTML file augmented with special
control codes. Because of this, you can have as much control of your site’s HTML code as you like.
Every page in your site has a **page type**. We will briefly talk about page types later, and go into much more detail
in tutorial two; right now all our pages will be of the page type *Page*. When rendering a page, SilverStripe will look
for a template file in the *tutorial/templates* folder, with the name `<PageType>`.ss - in our case *Page.ss*.
Open *themes/tutorial/templates/Page.ss*. It uses standard HTML with three exceptions: `<% base_tag %>`, *$Content* and
*$SilverStripeNavigator*. These template markers are processed by SilverStripe into HTML before being sent to your
browser.
`<% base_tag %>` is replaced with the HTML [base element](http://www.w3.org/TR/html401/struct/links.html#h-12.4). This
ensures the browser knows where to locate your site's images and css files.
*$Content* is replaced with the content of the page currently being viewed. This allows you to make all changes to
your site's content in the CMS.
*$SilverStripeNavigator* inserts the HTML for the navigator at the bottom of the page, which allows you to move
quickly between the CMS and the draft and published version of your page.

**Flushing the cache**
Whenever we edit a template file, we need to append *?flush=1* onto the end of the URL, e.g.
http://localhost/home/?flush=1. SilverStripe stores template files in a cache for quicker load times. Whenever there are
changes to the template, we must flush the cache in order for the changes to take effect.
## Inserting the page title
Let's introduce two new template variables - *$Title* and *$MetaTags*.
*$Title* is simply replaced with the name of the page ('Page name' on the 'Main' tab in the editor).
Open *themes/tutorial/templates/Page.ss* and find the following code:
:::ss
<div id="Header">
<h1> </h1>
</div>
and replace it with
:::ss
<div id="Header">
<h1>$Title</h1>
</div>
*$MetaTags* adds meta tags for search engines, as well as the page title ('Title' on the 'Meta-data' tab in the
editor). You can define your metatags in the meta-data tab off the content editor in the CMS.
Add *$MetaTags* to the head so that it looks like this:
:::ss
<head>
<% base_tag %>
$MetaTags
<% require themedCSS(layout) %>
<% require themedCSS(typography) %>
<% require themedCSS(form) %>
</head>
> Don't forget to flush the cache each time you change a template by adding *?flush=1* onto the end of the URL.
Your page should now look something like this (with your own content of course):

## Making a Navigation System
So far we have made several pages, but we have no way to navigate between them. We can create a menu for our site using
a **control block**. Control blocks allow us to iterate over a data set, and render each item using a sub-template. The
**page control** *Menu(1)* returns the set of the first level menu items. We can then use the template variable
*$MenuTitle* to show the title of the page we are linking to.
Open up *themes/tutorial/templates/Page.ss*, and insert the following code inside `<div id="Main">`:
:::ss
<ul id="Menu1">
<% control Menu(1) %>
<li><a href="#">$MenuTitle</a></li>
<% end_control %>
</ul>
Here we've created an unordered list called *Menu1*, which *themes/tutorial/css/layout.css* will style into the menu.
Then, using a control block over the page control *Menu(1)*, we add a link to the list for each menu item.
All going to plan, your page should look like this:

The menu isn't really very useful until each button links to the relevant page. We can get the link for the menu item in
question by using the *$Link* template variable.
Replace the list item line with this one:
:::ss
<li><a href="$Link" title="Go to the "{$Title}" page">$MenuTitle</a></li>
> $Title refers to *Page Name* in the CMS, whereas $MenuTitle refers to (the often shorter) *Navigation label*
## Highlighting the current page
A useful feature is highlighting the current page the user is looking at. We can do this with the template variable
*$LinkingMode*. *$LinkingMode* returns one of three values:
* *current* - This page is being visited, and should be highlighted
* *link* - The page is not currently being visited, so shouldn't be highlighted
* *section* - A page under this page is being visited so you probably want to highlight it.
> For example: if you were visiting a staff member such as "Home > Company > Staff > <NAME>", you would want to highlight 'Company' to say you are in that section.
Highlighting the current page is easy, simply assign a css class based on the value of *$LinkingMode*. Then provide a different style for current/section in css, as has been provided for you in *tutorial/css/layout.css*.
Change the list item line in *Page.ss* so it looks like this:
:::ss
<li class="$LinkingMode">
<a href="$Link" title="Go to the "{$Title}" page">$MenuTitle</a>
</li>
You should now have a fully functional top menu

## Adding a second level
Although we have a fully functional navigation system, it is currently quite restrictive. Currently there is no way to
nest pages, we have a completely flat site. Adding a second level in SilverStripe is easy. First, let's add some pages. The "About Us" section could use some expansion.
Select "About Us", and create two new pages "What we do" and "Our History" of the type "Page" inside. You can also create the pages elsewhere on the site tree, and use the reorganize button to drag and drop the pages into place.
Either way, your site tree should now look something like this:

Great, we now have a hierarchical site structure, but we still have no way of getting to these second level pages.
Adding a second level menu is very similar to adding the first level menu.
Open up our *Page.ss* template, and find the `<div id="ContentContainer">` tag. Underneath it, add the following code:
:::ss
<ul id="Menu2">
<% control Menu(2) %>
<li class="$LinkingMode"><a href="$Link" title="Go to the "{$Title}" page">$MenuTitle</a></li>
<% end_control %>
</ul>
This should look very familiar. It is exactly the same as our first menu, except we have named our linked list *Menu2*
and we are using the control *Menu(2)* instead of *Menu(1)*. As we can see here, the *Menu* control takes a single
argument - the level of the menu we want to get. Our css file will style this linked list into the second level menu,
using our usual *$LinkingMode* technique to highlight the current page.
We now have our second level menu, but we also have a problem. The menu is displayed on every page, even those that
don't have any nested pages. We can solve this problem with an **if block**. Simply surround the menu with an if block
like this:
:::ss
<% if Menu(2) %>
<ul id="Menu2">
<% control Menu(2) %>
<li class="$LinkingMode"><a href="$Link" title="Go to the "{$Title}" page">$MenuTitle</a></li>
<% end_control %>
</ul>
<% end_if %>
The if block only includes the code inside it if the condition is true. In this case, it checks for the existence of
*Menu(2)*. If it exists then the code inside will be processed and the menu will be shown. Otherwise the code will not
be processed and the menu will not be shown.
Now that we have two levels of navigation, it would also be useful to include some "breadcrumbs".
Find *`<div id="Content" class="typography">`* and underneath it add:
:::ss
<div class="breadcrumbs">
$Breadcrumbs
</div>
Breadcrumbs are only useful on pages that aren't in the top level. We can ensure that we only show them if we aren't in
the top level with another if statement.
:::ss
<% if Level(2) %>
<div class="breadcrumbs">
$Breadcrumbs
</div>
<% end_if %>
The *Level* page control allows you to get data from the page's parents, eg if you used *Level(1)*, you could use
*$Level(1).Title* to get the top level page title. In this case, we merely use it to check the existence of a second
level page; if one exists then we include the breadcrumbs.
We now have a fully functioning two level navigation system. Both menus should be updating and highlighting as you move
from page to page. They will also mirror changes done in the SilverStripe CMS, such as renaming pages or moving them
around.

## Using a different template for the home page
So far, a single template *Page.ss* is being used for the entire site. This is useful for the purpose of this
tutorial, but in a finished website we can expect there to be several page layouts.
To illustrate how we do this, we will create a new template for the homepage. This template will have a large graphical
banner to welcome visitors.
### Creating a new page type
Earlier we stated that every page in a SilverStripe site has a **page type**, and that SilverStripe will look for a
template corresponding to the page type. Therefore, the first step to get the homepage using a different template is to
create a new page type.
Each page type is represented by two php classes: a *data object* and a *controller*. Don't worry about the details of page
types right now, we will go into much more detail in tutorial two.
Create a new file *HomePage.php* in *mysite/code*. Copy the following code into it:
:::php
<?php
/**
* Defines the HomePage page type
*/
class HomePage extends Page {
static $db = array(
);
static $has_one = array(
);
}
class HomePage_Controller extends Page_Controller {
}
Every page type also has a database table corresponding to it. Every time we modify the database, we need to rebuild it.
We can do this by going to [http://localhost/dev/build?flush=1](http://localhost/dev/build?flush=1). It may take a
moment, so be patient. This add tables and fields needed by your site, and modifies any structures that have changed. It
does this non-destructively - it will never delete your data.
As we have just created a new page type, SilverStripe will add this to the list of page types in the database.
### Changing the page type of the Home page
After building the database, we can change the page type of the homepage in the CMS.
Under the "Behaviour" tab. Change it to *HomePage*, and click "Save Draft" and "Publish".

Our homepage is now of the page type *HomePage*. However, even though it is of the *HomePage* page type, it is still
rendered with the *Page* template. SilverStripe still renders the homepage using the *Page* template because when we
created the *HomePage* page type, we inherited from *Page*. So when SilverStripe cannot find a *HomePage* template, it
will use the *Page* template. SilverStripe always attempts to use the most specific template first, and then falls back
to the template of the page type's parents.
### Creating a new template
To create a new template, create a copy of *Page.ss* (found in *themes/tutorial/templates*) and call it *HomePage.ss*. If we flush the cache (*?flush=1*), SilverStripe should now be using *HomePage.ss* for the homepage, and *Page.ss* for the rest of the site. Now let's customize the *HomePage* template.
First, remove the breadcrumbs and the secondary menu; we don't need them for the homepage. Let's replace the title with our image. Add this line above the *Content* div:
:::ss
<div id="Banner">
<img src="themes/tutorial/images/welcome.png" alt="Homepage image" />
</div>

### Using a subtemplate
Having two templates is good, but we have a lot of identical code in the two templates. Rather than having two
completely separate templates, we can use subtemplates to specify only the part of the template that has changed.
If we compare *Page.ss* and *HomePage.ss*, we can see the only differences are within the *ContentContainer* div.
Copy the contents of the *ContentContainer* div from *themes/tutorial/templates/Page.ss* to a new file
*themes/tutorial/templates/Layout/Page.ss*, and do the same from *themes/tutorial/templates/HomePage.ss* to
*themes/tutorial/templates/Layout/HomePage.ss*.
The files should look like this:
**themes/tutorial/templates/Layout/Page.ss**
:::ss
<% if Menu(2) %>
<ul id="Menu2">
<% control Menu(2) %>
<li class="$LinkingMode"><a href="$Link" title="Go to the "{$Title}" page">$MenuTitle</a></li>
<% end_control %>
</ul>
<% end_if %>
<div id="Content" class="typography">
<% if Level(2) %>
<div class="breadcrumbs">
$Breadcrumbs
</div>
<% end_if %>
$Content
$Form
</div>
**themes/tutorial/templates/Layout/HomePage.ss**
:::ss
<div id="Banner">
<img src="themes/tutorial/images/welcome.png" alt="Homepage image" />
</div>
<div id="Content" class="typography">
$Content
</div>
We can then delete *themes/tutorial/templates/HomePage.ss*, as it is no longer needed.
Replace the code we just copied out of *themes/tutorial/templates/Page.ss* with *$Layout*, so it looks like this:
**themes/tutorial/templates/Page.ss**
:::ss
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.1//EN" "http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" >
<head>
<% base_tag %>
$MetaTags
<% require themedCSS(layout) %>
<% require themedCSS(typography) %>
<% require themedCSS(form) %>
</head>
<body>
<div id="Main">
<ul id="Menu1">
<% control Menu(1) %>
<li class="$LinkingMode"><a href="$Link" title="Go to the "{$Title}" page">$MenuTitle</a></li>
<% end_control %>
</ul>
<div id="Header">
<h1>$Title</h1>
</div>
<div id="ContentContainer">
$Layout
</div>
<div id="Footer">
<span>Visit <a href="http://www.silverstripe.com" title="Visit www.silverstripe.com">www.silverstripe.com</a> to download the CMS</span>
</div>
</div>
$SilverStripeNavigator
</body>
</html>
> As you have just done a few template changes, remember to add *?flush=1* in your URL to flush the cache so see your changes in the browser
SilverStripe first searches for a template in the *themes/tutorial/templates* folder. Since there is no *HomePage.ss*,
it will use the *Page.ss* for both *Page* and *HomePage* page types. When it comes across the *$Layout* tag, it will
then descend into the *themes/tutorial/templates/Layout* folder, and will use *Page.ss* for the *Page* page type, and
*HomePage.ss* for the *HomePage* page type.

## Summary
Starting from a basic template, we have introduced template variables, controls and if blocks, and we have used these
to build a basic but fully functional site. You have also been briefly introduced to page types, and seen how they
correspond to templates and subtemplates. By using these templates, you have seen how to customize the site content
according to the page type of the page you are displaying.
In the next tutorial, [Extending a Basic Site](2-extending-a-basic-site), we will explore page types on a
deeper level, and see how you can customize your own page types to extend SilverStripe to do much more interesting
things.
[Next Tutorial >>](2-extending-a-basic-site)
## Books on SilverStripe
* [Official book on SilverStripe in English](http://www.silverstripe.org/silverstripe-book).
* [Official book on SilverStripe in German](http://www.silverstripe.org/das-silverstripe-buch).

<file_sep>/docs/en/tutorials/2-extending-a-basic-site.md
# Tutorial 2 - Extending a basic site
## Overview
In the [first tutorial](1-building-a-basic-site) we learned how to create a basic site using SilverStripe. This
tutorial builds on what you have learned in [the first tutorial](1-building-a-basic-site), so it is recommended
that you complete it first.
In this tutorial you will explore extending SilverStripe by creating your own page types. In doing this you will get a
good overview of how SilverStripe works.
## What are we working towards?
Throughout this tutorial we are going to work on adding two new sections to the site we built in the first tutorial. The
first is a news section, with a recent news listing on the homepage and an RSS feed. The second is a staff section,
which demonstrates more complex database structures by associating an image with each staff member.

## The SilverStripe data model
A large part of designing complex SilverStripe sites is the creation of your own page types. Before we progress any
further, it is important to understand what a page type is, and how the SilverStripe data model works.
SilverStripe is based on the **"Model-View-Controller"** design pattern. This means that SilverStripe attempts to separate
data, logic and presentation as much as possible. Every page has three separate parts which are combined to give you the
final page. Lets look at each one individually:
### Model
All content on your site is stored in a database. There is a table in the database corresponding for every class that is
a child of the `[api:DataObject]` class. Every object of that class corresponds to a row in that table -
this is your "data object", the **"model"** of Model-View-Controller. A page type has a data object that represents all the data for your page - rather than inheriting
directly from data object it inherits from `[api:SiteTree]`. We generally create a "Page" data object, and subclass this for
the rest of the page types. This allows us to define behavior that is consistent across all pages in our site.
### View
The **"view"** is the presentation of your site. As we have already seen, the templates SilverStripe uses to render a page
is dependent on the page type. Using both your templates and css, you are able to have full control over the
presentation of your site.
### Controller
A page type also has a **"controller"**. A controller contains all the code used to manipulate your data before it is
rendered. For example, suppose you were making an auction site, and you only wanted to display the auctions closing in
the next ten minutes. You would implement this in the controller. The controller for a page should inherit from
`[api:ContentController]`. Just as we create a "Page" data object and subclass it for the rest of the
site, we also create a "Page_Controller" that is subclassed.
Creating a new page type simply requires creating these three things. You can then have full control over presentation,
the database, which fields can be edited in the CMS, and can use code to make our pages do much more clever things.
A more in-depth introduction of Model-View-Controller can be found
[here](http://www.slash7.com/articles/2005/02/22/mvc-the-most-vexing-conundrum).

## Creating the news section page types
Let's make our news section. We'll need two new page types for this. The first one is obvious: we need an *ArticlePage*
page type. The second is a little less obvious: we need an *ArticleHolder* page type that contains our articles.
We'll start with the *ArticlePage* page type. First we create the model, a class called "ArticlePage". We put the
*ArticlePage* class into a file called "ArticlePage.php" inside *mysite/code*. We also put the controller,
*ArticlePage_Controller*, in here. Any other classes that are related to *ArticlePage* – for example, the class
*ArticlePage_AnythingElse* - will also go into "ArticlePage.php".
**mysite/code/ArticlePage.php**
:::php
<?php
/**
* Defines the ArticlePage page type
*/
class ArticlePage extends Page {
static $db = array(
);
static $has_one = array(
);
}
class ArticlePage_Controller extends Page_Controller {
}
Here we've created our data object/controller pair, but we haven't actually extended them at all. Don't worry about the
*$db* and *$has_one* arrays just yet, we'll explain them soon, as well as other ways in which you can extend your page
types. SilverStripe will use the template for the *Page* page type as explained in the first tutorial, so we don't need
to specifically create the view for this page type.
Let's create the *ArticleHolder* page type.
**mysite/code/ArticleHolder.php**
:::php
<?php
/**
* Defines the ArticleHolder page type
*/
class ArticleHolder extends Page {
static $db = array(
);
static $has_one = array(
);
static $allowed_children = array('ArticlePage');
}
class ArticleHolder_Controller extends Page_Controller {
}
Here we have done something interesting: the *$allowed_children* field. This is one of a number of static fields we can
define to change the properties of a page type. The *$allowed_children* field is an array of page types that are allowed
to be children of the page in the site tree. As we only want news articles in the news section, we only want
*ArticlePage* pages for children. We can enforce this in the CMS by setting the *$allowed_children* field.
We will be introducing other fields like this as we progress; there is a full list in the documentation for
`[api:SiteTree]`.
Now that we have created our page types, we need to let SilverStripe rebuild the database. If we rebuild the database by
going to [http://localhost/dev/build?flush=1](http://localhost/dev/build?flush=1), SilverStripe will detect that there are two
new page types and add them to the list of page types in the database.
> It is SilverStripe convention to suffix general page types with "Page", and page types that hold other page types with
> "Holder". This is to ensure that we don't have URLs with the same name as a page type; if we named our *ArticleHolder*
> page type "News", it would conflict with the page name also called "News".
## Adding date and author fields
Now that we have an *ArticlePage* page type, let's make it a little more useful. Remember the *$db* array? We can use
this array to add extra fields to the database. It would be nice to know when each article was posted, and who posted
it. Change the *$db* array in the *ArticlePage* class so it looks like this:
:::php
<?php
class ArticlePage extends Page {
static $db = array(
'Date' => 'Date',
'Author' => 'Text'
);
// .....
}
Every entry in the array is a key-value pair. The key is the name of the field, and the value is the type. We have a
`[api:Date]` for a complete list of different data types.
> Note: The names chosen for the fields you add must not already be used. Be careful using field names such as Title,
> Content etc. as these may already be defined in the page types your new page is extending from.
If we rebuild the database, we will see that now the *ArticlePage* table is created. Even though we had an *ArticlePage*
page type before, the table was not created because we had no fields that were unique to the article page type. We now
have the extra fields in the database, but still no way of changing them. To add these fields to the CMS we have to
override the *getCMSFields()* method, which is called by the CMS when it creates the form to edit a page. Add the
method to the *ArticlePage* class.
:::php
<?php
class ArticlePage extends Page {
// ...
public function getCMSFields() {
$fields = parent::getCMSFields();
$fields->addFieldToTab('Root.Content', new DateField('Date'), 'Content');
$fields->addFieldToTab('Root.Content', new TextField('Author'), 'Content');
return $fields;
}
}
// ...
Let's walk through this method.
:::php
$fields = parent::getCMSFields();
Firstly, we get the fields from the parent class; we want to add fields, not replace them. The *$fields* variable
returned is a `[api:FieldList]` object.
:::php
$fields->addFieldToTab('Root.Content', new DateField('Date'), 'Content');
$fields->addFieldToTab('Root.Content', new TextField('Author'), 'Content');
We can then add our new fields with *addFieldToTab*. The first argument is the tab on which we want to add the field to:
"Root.Content" is the tab which the content editor is on. The second argument is the field to add; this is not a
database field, but a `[api:FormField]` documentation for more details.
:::php
return $fields;
Finally, we return the fields to the CMS. If we flush the cache (by adding ?flush=1 at the end of the URL), we will be able
to edit the fields in the CMS.
Now that we have created our page types, let's add some content. Go into the CMS and create an *ArticleHolder* page
named "News", and create some *ArticlePage*s inside it.

## Modifing the date field
**Please note:** As of version 2.4, the DateField type no longer automatically adds a javascript datepicker. Your date field will look just like a text field.
This makes it confusing and doesn't give the user much help when adding a date.
To make the date field a bit more user friendly, you can add a dropdown calendar, set the date format and add better title.
:::php
<?php
class ArticlePage extends Page {
// .....
public function getCMSFields() {
$fields = parent::getCMSFields();
$fields->addFieldToTab('Root.Content', $dateField = new DateField('Date','Article Date (for example: 20/12/2010)'), 'Content');
$dateField->setConfig('showcalendar', true);
$dateField->setConfig('dateformat', 'dd/MM/YYYY');
$fields->addFieldToTab('Root.Content', new TextField('Author','Author Name'), 'Content');
return $fields;
}
Let's walk through these changes.
:::php
$fields->addFieldToTab('Root.Content', $dateField = new DateField('Date','Article Date (for example: 20/12/2010)'), 'Content');
*$dateField* is added only to the DateField in order to change the configuration.
:::php
$dateField->setConfig('showcalendar', true);
Set *showCalendar* to true to have a calendar appear underneath the Date field when you click on the field.
:::php
$dateField->setConfig('dateformat', 'dd/MM/YYYY');
*dateFormat* allows you to specify how you wish the date to be entered and displayed in the CMS field.
:::php
$fields->addFieldToTab('Root.Content', new TextField('Author','Author Name'), 'Content');
By default the first argument *'Date'* or *'Author'* is shown as the title, however this might not be that helpful so to change the title,
add the new title as the second argument. See the `[api:DateField]` documentation for more details.
## Creating the templates
We can already look at the content of news pages on our site, because the article holder page and the article pages
inherit their templates from Page. But we're not getting the author and date fields displayed in either case.
So let's create a template for each of our new page types. We'll put these in *themes/tutorial/templates/Layout* so we
only have to define the page specific parts: SilverStripe will use *themes/tutorial/templates/Page.ss* for the basic
page layout.
First, the template for displaying a single article:
**themes/tutorial/templates/Layout/ArticlePage.ss**
:::ss
<% if Menu(2) %>
<ul id="Menu2">
<% control Menu(2) %>
<li class="$LinkingMode"><a href="$Link" title="Go to the $Title page">$MenuTitle</a></li>
<% end_control %>
</ul>
<% end_if %>
<div id="Content" class="typography">
<% if Level(2) %>
<div class="breadcrumbs">
$Breadcrumbs
</div>
<% end_if %>
<h1>$Title</h1>
$Content
<div class="newsDetails">
Posted on $Date.Nice by $Author
</div>
</div>
The first block of code is our regular second level menu; we also have our regular breadcrumbs code here. We will see
how to remove these blocks of repetitive code in a bit.
We use *$Date* and *$Author* to access the new fields. In fact, all template variables and page controls come from
either the data object or the controller for the page being displayed. The *$Breadcrumbs* variable comes from the
*Breadcrumbs()* method of the `[api:SiteTree]` class. *$Date* and *$Author* come from the *Article* table through
your data object. *$Content* comes from the *SiteTree* table through the same data object. The data for your page is
spread across several tables in the database matched by id - e.g. *Content* is in the *SiteTree* table, and *Date* and
*Author* are in the *Article* table. SilverStripe matches these records by their ids and collates them into the single
data object.

Rather than using *$Date* directly, we use *$Date.Nice*. If we look in the `[api:Date]` documentation, we can see
that the *Nice* function returns the date in *dd/mm/yyyy* format, rather than the *yyyy-mm-dd* format stored in the
database.

Now we'll create a template for the article holder: we want our news section to show a list of news items, each with a
summary.
**themes/tutorial/templates/Layout/ArticleHolder.ss**
:::ss
<div id="Content" class="typography">
$Content
<ul id="NewsList">
<% control Children %>
<li class="newsDateTitle"><a href="$Link" title="Read more on "{$Title}"">$Title</a></li>
<li class="newsDateTitle">$Date.Nice</li>
<li class="newsSummary">$Content.FirstParagraph <a href="$Link" title="Read more on "{$Title}"">Read more >></a></li>
<% end_control %>
</ul>
</div>
Here we use the page control *Children*. As the name suggests, this control allows you to iterate over the children of a
page, which in this case is our news articles. The *$Link* variable will give the address of the article which we can
use to create a link, and the *FirstParagraph* function of the `[api:HTMLText]` field gives us a nice summary of the
article.

Remember that the visual styles are not part of the CMS, they are defined in the tutorial CSS file.
### Using include files in templates
The second level menu is something we want in most, but not all, pages so we can't put it in the base template. By
putting it in a separate file in the *tutorial/templates/Includes* folder, we can use `<% include templatename %>` to
include it in our other templates. Separate the second level menu into a new file *themes/tutorial/templates/Includes/Menu2.ss*.
**themes/tutorial/templates/Includes/Menu2.ss**
:::ss
<% if Menu(2) %>
<ul id="Menu2">
<% control Menu(2) %>
<li class="$LinkingMode"><a href="$Link" title="Go to the $Title page">$MenuTitle</a></li>
<% end_control %>
</ul>
<% end_if %>
And then replace the second level menu with `<% include Menu2 %>` in *Page.ss* and *ArticlePage.ss* like so:
**themes/tutorial/templates/Layout/Page.ss**, **themes/tutorial/templates/Layout/ArticlePage.ss**
:::ss
<% include Menu2 %>
<div id="Content" class="typography">
...
Do the same with the breadcrumbs:
**themes/tutorial/templates/Includes/Breadcrumbs.ss**
:::ss
<% if Level(2) %>
<div class="breadcrumbs">
$Breadcrumbs
</div>
<% end_if %>
**themes/tutorial/templates/Layout/Page.ss**, **themes/tutorial/templates/Layout/ArticlePage.ss**
:::ss
...
<div id="Content" class="typography">
<% include Breadcrumbs %>
...
You can make your templates more modular and easier to maintain by separating commonly-used pieces into include files.
### Changing the icons of pages in the CMS
Let's now make a purely cosmetic change that nevertheless helps to make the information presented in the CMS clearer.
Add the following field to the *ArticleHolder* and *ArticlePage* classes:
:::php
static $icon = "themes/tutorial/images/treeicons/news";
And this one to the *HomePage* class:
:::php
static $icon = "themes/tutorial/images/treeicons/home";
This will change the icons for the pages in the CMS.
> Note: that the corresponding filename to the path given for $icon will end with **-file.gif**,
> e.g. when you specify **news** above, the filename will be **news-file.gif**.

## Showing the latest news on the homepage
It would be nice to greet page visitors with a summary of the latest news when they visit the homepage. This requires a
little more code though - the news articles are not direct children of the homepage, so we can't use the *Children*
control. We can get the data for the news articles by implementing our own function in *HomePage_Controller*.
**mysite/code/HomePage.php**
:::php
...
public function LatestNews($num=5) {
$news = DataObject::get_one("ArticleHolder");
return ($news) ? DataObject::get("ArticlePage", "ParentID = $news->ID", "Date DESC", "", $num) : false;
}
...
This function simply runs a database query that gets the latest news articles from the database. By default, this is
five, but you can change it by passing a number to the function. See the `[api:DataObject]` documentation for
details. We can reference this function as a page control in our *HomePage* template:
**themes/tutorial/templates/Layout/Homepage.ss**
:::ss
...
$Content
<ul id="NewsList">
<% control LatestNews %>
<li class="newsDateTitle"><a href="$Link" title="Read more on "{$Title}"">$Title</a></li>
<li class="newsDateTitle">$Date.Nice</li>
<li class="newsSummary">$Content.FirstParagraph<a href="$Link" title="Read more on "{$Title}"">Read more >></a></li>
<% end_control %>
</ul>
...
When SilverStripe comes across a variable or page control it doesn't recognize, it first passes control to the
controller. If the controller doesn't have a function for the variable or page control, it then passes control to the
data object. If it has no matching functions, it then searches its database fields. Failing that it will return nothing.
The controller for a page is only created when page is actually visited, while the data object is available when the
page is referenced in other pages, e.g. by page controls. A good rule of thumb is to put all functions specific to the
page currently being viewed in the controller; only if a function needs to be used in another page should you put it in
the data object.

## Creating a RSS feed
An RSS feed is something that no news section should be without. SilverStripe makes it easy to create RSS feeds by
providing an `[api:RSSFeed]` class to do all the hard work for you. Create the following function in the
*ArticleHolder_Controller*:
:::php
public function rss() {
$rss = new RSSFeed($this->Children(), $this->Link(), "The coolest news around");
$rss->outputToBrowser();
}
This function simply creates an RSS feed of all the news articles, and outputs it to the browser. If you go to
[http://localhost/news/rss](http://localhost/news/rss) you will see our RSS feed. What happens here is that
when there is more to a URL after the page's base URL - "rss" in this case - SilverStripe will call the function with
that name on the controller if it exists.
Depending on your browser, you should see something like the picture below. If your browser doesn't support RSS, you
will most likely see the XML output instead.

Now all we need is to let the user know that our RSS feed exists. The `[api:RSSFeed]` in your controller, it will be
called when the page is requested. Add this function to *ArticleHolder_Controller*:
:::php
public function init() {
RSSFeed::linkToFeed($this->Link() . "rss");
parent::init();
}
This automatically generates a link-tag in the header of our template. The *init* function is then called on the parent
class to ensure any initialization the parent would have done if we hadn't overridden the *init* function is still
called. In Firefox you can see the RSS feed link in the address bar:

## Adding a staff section
Now that we have a complete news section, let's move on to the staff section. We need to create *StaffHolder* and
*StaffPage* page types, for an overview on all staff members and a detail-view for a single member. First let's start
with the *StaffHolder* page type.
**mysite/code/StaffHolder.php**
:::php
<?php
class StaffHolder extends Page {
static $db = array(
);
static $has_one = array(
);
static $allowed_children = array('StaffPage');
}
class StaffHolder_Controller extends Page_Controller {
}
Nothing here should be new. The *StaffPage* page type is more interesting though. Each staff member has a portrait
image. We want to make a permanent connection between this image and the specific *StaffPage* (otherwise we could simply
insert an image in the *$Content* field).
**mysite/code/StaffPage.php**
:::php
<?php
class StaffPage extends Page {
static $db = array(
);
static $has_one = array(
'Photo' => 'Image'
);
public function getCMSFields() {
$fields = parent::getCMSFields();
$fields->addFieldToTab("Root.Content.Images", new UploadField('Photo'));
return $fields;
}
}
class StaffPage_Controller extends Page_Controller {
}
Instead of adding our *Image* as a field in *$db*, we have used the *$has_one* array. This is because an *Image* is not
a simple database field like all the fields we have seen so far, but has its own database table. By using the *$has_one*
array, we create a relationship between the *StaffPage* table and the *Image* table by storing the id of the respective
*Image* in the *StaffPage* table.
We then add an `[api:UploadField]` in the *getCMSFields* function to the tab "Root.Content.Images". Since this tab doesn't exist,
the *addFieldToTab* function will create it for us. The *ImageField* allows us to select an image or upload a new one in
the CMS.

Rebuild the database ([http://localhost/dev/build?flush=1](http://localhost/dev/build?flush=1)) and open the CMS. Create
a new *StaffHolder* called "Staff" in the "About Us" section, and create some *StaffPage*s in it.

### Creating the staff section templates
The staff section templates aren't too difficult to create, thanks to the utility methods provided by the `[api:Image]` class.
**themes/tutorial/templates/Layout/StaffHolder.ss**
:::ss
<% include Menu2 %>
<div id="Content" class="typography">
<% include Breadcrumbs %>
$Content
<ul id="StaffList">
<% control Children %>
<li>
<div class="staffname"><a href="$Link">$Title</a></div>
<div class="staffphoto">$Photo.SetWidth(50)</div>
<div class="staffdescription"><p>$Content.FirstSentence</p></div>
</li>
<% end_control %>
</ul>
</div>
This template is very similar to the *ArticleHolder* template. The *SetWidth* method of the `[api:Image]` class
will resize the image before sending it to the browser. The resized image is cached, so the server doesn't have to
resize the image every time the page is viewed.

The *StaffPage* template is also very straight forward.
**themes/tutorial/templates/Layout/StaffPage.ss**
:::ss
<% include Menu2 %>
<div id="Content" class="typography">
<% include Breadcrumbs %>
<div id="StaffPhoto">
$Photo.SetWidth(150)
</div>
$Content
</div>
Here we also use the *SetWidth* function to get a different sized image from the same source image. You should now have
a complete staff section.

## Summary
In this tutorial we have explored the concept of page types. In the process of creating and extending page types you
have been introduced to many of the concepts required to build a site with SilverStripe.
[Next Tutorial >>](3-forms)
<file_sep>/forms/gridfield/GridFieldToolbarHeader.php
<?php
/**
* Adding this class to a {@link GridFieldConfig} of a {@link GridField} adds a header title to that field.
* The header serves double duty of displaying the name of the data the GridField is showing and
* providing a space for actions on this grid.
*
* This header provides two new HTML fragment spaces: 'toolbar-header-left' and 'toolbar-header-right'.
*
* @package framework
* @subpackage gridfield
*/
class GridFieldToolbarHeader implements GridField_HTMLProvider {
public function getHTMLFragments( $gridField) {
return array(
'header' => $gridField->renderWith('GridFieldToolbarHeader')
);
}
}
<file_sep>/docs/en/topics/page-type-templates.md
# Building templates for page types
Much of your work building a SilverStripe site will involve the creation of templates for your [page types](/topics/page-types). SilverStripe has its own template language, which is described in full [here](/reference/templates).
SilverStripe templates consist of HTML code augmented with special control codes, described below. Because of this, you can have as much control of your site's HTML code as you like.
Take a look at mysite/templates/Page.ss. It contains standard HTML markup, with some extra tags. You can see that this file only generates some of the content – it sets up the `<html>` tags, deals with the `<head>` section, creates the first-level navigation, and then closes it all off again. See `$Layout`? That’s what is doing most of the work when you visit a page.
Now take a look at `mysite/templates/Layout/Page.ss`. This as you can see has a lot more markup in it – it’s what is included into `$Layout` when the ‘Page’ page type is rendered. Similarly, `mysite/templates/Layout/HomePage.ss` would be rendered into `$Layout` when the ‘HomePage’ page type is selected for the current page you’re viewing.
Here is a very simple pair of templates. We shall explain their contents item by item.
`templates/Page.ss`
:::ss
<html>
<%-- This is my first template --%>
<head>
<% base_tag %>
<title>$Title</title>
$MetaTags
</head>
<body>
<div id="Container">
<div id="Header">
<h1><NAME></h1>
<% with $CurrentMember %>
<p>You are logged in as $FirstName $Surname.</p>
<% end_with %>
</div>
<div id="Navigation">
<% if $Menu(1) %>
<ul>
<% loop $Menu(1) %>
<li><a href="$Link" title="Go to the $Title page" class="$LinkingMode">$MenuTitle</a></li>
<% end_loop %>
</ul>
<% end_if %>
</div>
<div class="typography">
$Layout
</div>
<div id="Footer">
<p>Copyright $Now.Year</p>
</div>
</div>
</body>
</html>
`templates/Layout/Page.ss`
<h1>$Title</h1>
$Content
$Form
## <%-- This is my first template --%>
This is a comment. Like HTML comments, these tags let you include explanatory information in your comments. Unlike HTML comments, these tags won't be included in the HTML file downloaded by your visitors.
## <% base_tag %>
This tag must always appear in the `<head>` of your templates. SilverStripe uses a combination of a site-wide base tag and relative links to ensure that a site can function when loaded into a subdirectory on your webserver, as this is handy when developing a site. For more information see the [templates reference](/reference/templates#base-tag)
### $MetaTags
The `$MetaTags` placeholder in a template returns a segment of HTML appropriate for putting into the `<head>` tag. It
will set up title, keywords and description meta-tags, based on the CMS content and is editable in the 'Meta-data' tab
on a per-page basis. If you don’t want to include the title-tag `<title>` (for custom templating), use
`$MetaTags(false)`.
By default `$MetaTags` renders:
:::ss
<title>Title of the Page</title>
<meta name="generator" http-equiv="generator" content="SilverStripe 2.0" >
<meta http-equiv="Content-type" content="text/html; charset=utf-8" >
### <% with $CurrentMember %>...<% end_with %>
This is a "with" block. A with block will change the "scope" of the template, so that all template variables inside that block will contain values from the $CurrentMember object, rather than from the page being rendered.
`$CurrentMember` is an object with information about the currently logged in member. If no-one is logged in, then it's blank. In that case, the entire `<% with $CurrentMember %>` block will be omitted.
### $FirstName, $Surname
These two variables come from the `$CurrentMember` object, because they are inside the `<% with $CurrentMember %>` block. In particular, they will contain the first name and surname of the currently logged in member.
### <% if $Menu(1) %>...<% end_if %>
This template code defines a conditional block. Its contents will only be shown if `$Menu(1)` contains anything.
`$Menu(1)` is a built-in page control that defines the top-level menu. You can also create a sub-menu using `$Menu(2)`, and a third-level menu using using `$Menu(3)`, etc.
### <% loop $Menu(1) %>...<% end_loop %> %>
This template code defines a repeating element. `$Menu(1)`. Like `<% with %>`, the loop block will change the "scope" of your template, which means that all of the template variables you use inside it will refer to a menu item. The template code will be repeated once per menu item, with the scope set to that menu item's page.
### $Link, $Title, $MenuTitle
Because these 3 variables are within the repeating element, then their values will come from that repeating element. In this case, they will be the values of each menu item.
* `$Link`: A link to that menu item.
* `$Title`: The page title of that menu item.
* `$MenuTitle`: The navigation label of that menu item.
### $LinkingMode
Once again, this is a variable that will be source from the menu item. This variable differs for each menu item, and will be set to one of these 3 values:
* `link`: You are neither on this page nor in this section.
* `current`: You are currently on this page.
* `section`: The current page is a child of this menu item; so this is menu item identifies the section that you're currently in.
By setting the HTML class to this value, you can distinguish the styling of the currently selected menu item.
### $Layout
This variable will be replaced with the the rendered version of `templates/Layout/Page.ss`. If you create a page type that is a subclass of Page, then it is possible to only define `templates/Layout/MySubclassPage.ss`. In that case, then the rendered version of `templates/Layout/MySubclassPage.ss` wil be inserted into the `$Layout` variable in `templates/Page.ss`. This is a good way of defining a single main template and page specific sub-templates.
### $Now.Year
This will return the current year. `$Now` returns an `SS_Datetime` object, which has a number of methods, such as `Year`. See [the API documentation](api:SS_Datetime) for a list of all the methods.
### $Title
This is the same template code as used in the title attribute of your navgation. However, because we are using it outside of the `<% loop Menu(1) >` block, it will return the title of the current page, rather than the title of the menu item. We use this to make our main page title.
### $Content
This variable contains the content of the current page.
### $Form
Very often, a page will contain some content and a form of some kind. For example, the log-in page has a log-in form. If you are on such a page, this variable will contain the HTML content of the form. Putting it just below $Content is a good default.<file_sep>/docs/en/topics/data-types.md
# Data Types
These are the data-types that you can use when defining your data objects. They are all subclasses of `[api:DBField]`
for introducing their usage.
## Types
* `[api:Varchar]`: A variable-length string of up to 255 characters, designed to store raw text
* `[api:Text]`: A variable-length string of up to 2 megabytes, designed to store raw text
* `[api:HTMLVarchar]`: A variable-length string of up to 255 characters, designed to store HTML
* `[api:HTMLText]`: A variable-length string of up to 2 megabytes, designed to store HTML
* `[api:Enum]`: An enumeration of a set of strings
* `[api:Boolean]`: A boolean field.
* `[api:Int]`: An integer field.
* `[api:Decimal]`: A decimal number.
* `[api:Currency]`: A number with 2 decimal points of precision, designed to store currency values.
* `[api:Percentage]`: A decimal number between 0 and 1 that represents a percentage.
* `[api:Date]`: A date field
* `[api:SS_Datetime]`: A date / time field
* `[api:Time]`: A time field
## HTMLText vs. Text, and HTMLVarchar vs. Varchar
The database field types `[api:HTMLVarchar]` and `[api:Varchar]` are exactly the same in the database. However, the
templating engine knows to escape the `[api:Varchar]` field and not the `[api:HTMLVarchar]` field. So, it's important you
use the right field if you don't want to be putting $FieldType.XML everywhere.
If you're going to put HTML content into the field, please use the field type with the HTML prefix. Otherwise, you're
going to risk double-escaping your data, forgetting to escape your data, and generally creating a confusing situation.
## Usage
* See [datamodel](/topics/datamodel) for information about **database schemas** implementing these types
<file_sep>/control/PjaxResponseNegotiator.php
<?php
/**
* Handle the X-Pjax header that AJAX responses may provide, returning the
* fragment, or, in the case of non-AJAX form submissions, redirecting back to the submitter.
*
* X-Pjax ensures that users won't end up seeing the unstyled form HTML in their browser
* If a JS error prevents the Ajax overriding of form submissions from happening.
* It also provides better non-JS operation.
*
* Caution: This API is volatile, and might eventually be replaced by a generic
* action helper system for controllers.
*/
class PjaxResponseNegotiator {
/**
* @var Array See {@link respond()}
*/
protected $callbacks = array(
// TODO Using deprecated functionality, but don't want to duplicate Controller->redirectBack()
'default' => array('Director', 'redirectBack'),
);
/**
* @param RequestHandler $controller
* @param Array $callbacks
*/
function __construct($callbacks = array()) {
$this->callbacks = $callbacks;
}
/**
* Out of the box, the handler "CurrentForm" value, which will return the rendered form.
* Non-Ajax calls will redirect back.
*
* @param SS_HTTPRequest $request
* @param array $extraCallbacks List of anonymous functions or callables returning either a string
* or SS_HTTPResponse, keyed by their fragment identifier. The 'default' key can
* be used as a fallback for non-ajax responses.
* @return SS_HTTPResponse
*/
public function respond(SS_HTTPRequest $request, $extraCallbacks = array()) {
// Prepare the default options and combine with the others
$callbacks = array_merge(
array_change_key_case($this->callbacks, CASE_LOWER),
array_change_key_case($extraCallbacks, CASE_LOWER)
);
if($fragment = $request->getHeader('X-Pjax')) {
$fragment = strtolower($fragment);
if(isset($callbacks[$fragment])) {
return call_user_func($callbacks[$fragment]);
} else {
throw new SS_HTTPResponse_Exception("X-Pjax = '$fragment' not supported for this URL.", 400);
}
} else {
if($request->isAjax()) throw new SS_HTTPResponse_Exception("Ajax requests to this URL require an X-Pjax header.", 400);
return call_user_func($callbacks['default']);
}
}
/**
* @param String $fragment
* @param Callable $callback
*/
public function setCallback($fragment, $callback) {
$this->callbacks[$fragment] = $callback;
}
}
<file_sep>/docs/en/tutorials/5-dataobject-relationship-management.md
# Tutorial 5 - Dataobject Relationship Management
## Overview
In the [second tutorial](2-extending-a-basic-site) we have learned how to add extrafields to a page type thanks
to the *$db* array and how to add an image using the *$has_one* array and so create a relationship between a table and
the *Image* table by storing the id of the respective *Image* in the first table. This tutorial explores all this
relations between [DataObjects](/topics/datamodel#relations) and the way to manage them easily.
<div class="notice" markdown='1'>
I'm using the default tutorial theme in the following examples so the templates may vary or you may need to change
the template code in this example to fit your theme
</div>
## What are we working towards?
To simulate these relations between objects, we are going to simulate the management via the CMS of the **[Google Summer
Of Code 2007](http://www.silverstripe.com/google-summer-of-code-2007-we-are-in/)** that SilverStripe was part of.
To do this, we are gonna use the following objects :
* Project : Project on SilverStripe system for the GSOC
* Student : Student involved in the project
* Mentor : SilverStripe developer
* Module : Module used for the project
This is a table which sums up the relations between them :
| Project | Student | Mentor | Modules |
| ------- | ------- | ------ | ------------------
| i18n Multi-Language | <NAME> | <NAME> | Cms, Framework, i18n, Translation |
| Image Manipulation | <NAME> | <NAME> | Cms, Framework, ImageManipulation |
| Google Maps | <NAME> | <NAME> | Cms, Framework, Maps |
| Mashups | <NAME> | <NAME> | Cms, Framework, MashUps |
| Multiple Databases | <NAME> | <NAME> | Cms, Framework, MultipleDatabases |
| Reporting | <NAME> | <NAME> | Cms, Framework, Reporting |
| Security & OpenID | <NAME> | <NAME> | Cms, Framework, auth_openid |
| SEO | <NAME> | <NAME> | Cms, Framework, googleadwords, googleanalytics |
| Usability | <NAME> | <NAME> | Cms, Framework, UsabilityElijah |
| Safari 3 Support | <NAME> | <NAME> | Cms, Framework, UsabilityMeg |
## GSOC Projects
Before starting the relations management, we need to create a *ProjectsHolder* class where we will save the GSOC Project
pages.
*tutorial/code/ProjectsHolder.php*
:::php
<?php
class ProjectsHolder extends Page {
static $allowed_children = array( 'Project' );
}
class ProjectsHolder_Controller extends Page_Controller {
}
## Project - Student relation
**A project can only be done by one student.**
**A student has only one project.**
This relation is called a **1-to-1** relation.
The first step is to create the student and project objects.
*tutorial/code/Student.php*
:::php
<?php
class Student extends DataObject {
static $db = array(
'FirstName' => 'Text',
'Lastname' => 'Text',
'Nationality' => 'Text'
);
public function getCMSFields_forPopup() {
$fields = new FieldList();
$fields->push( new TextField( 'FirstName', 'First Name' ) );
$fields->push( new TextField( 'Lastname' ) );
$fields->push( new TextField( 'Nationality' ) );
return $fields;
}
}
*tutorial/code/Project.php*
:::php
<?php
class Project extends Page {
static $has_one = array(
'MyStudent' => 'Student'
);
}
class Project_Controller extends Page_Controller {}
This code will create a relationship between the *Project* table and the *Student* table by storing the id of the
respective *Student* in the *Project* table.
The second step is to add the table in the method *getCMSFields* which will allow you to manage the *has_one* relation.
:::php
class Project extends Page {
...
public function getCMSFields() {
$fields = parent::getCMSFields();
$tablefield = new HasOneComplexTableField(
$this,
'MyStudent',
'Student',
array(
'FirstName' => '<NAME>',
'Lastname' => '<NAME>',
'Nationality' => 'Nationality'
),
'getCMSFields_forPopup'
);
$tablefield->setParentClass('Project');
$fields->addFieldToTab( 'Root.Student', $tablefield );
return $fields;
}
}
Let’s walk through the parameters of the *HasOneComplexTableField* constructor.
1. **$this** : The first object concerned by the relation
2. **'MyStudent'** : The name of the second object of the relation
3. **'Student'** : The type of the second object of the relation
4. **array(...)** : The fields of the second object which will be in the table
5. **'getCMSFields_forPopup'** : The method which will be called to add, edit or only show a second object
You can also directly replace the last parameter by this code :
:::php
new FieldList(
new TextField( 'FirstName', 'First Name' ),
new TextField( 'Lastname' ),
new TextField( 'Nationality' )
);
<div class="tip" markdown='1'>
Don't forget to rebuild the database using *dev/build?flush=1* before you
proceed to the next part of this tutorial.
</div>
Now that we have created our *Project* page type and *Student* data object, let’s add some content.
Go into the CMS and create one *Project* page for each project listed [above](#what-are-we-working-towards) under a
*ProjectsHolder* page named **GSOC Projects** for instance.

As you can see in the tab panel *Student*, the adding functionality is titled *Add Student*. However, if you want to
modify this title, you have to add this code in the *getCMSFields* method of the *Project* class :
:::php
$tablefield->setAddTitle( 'A Student' );
Select now one of the *Project* page that you have created, go in the tab panel *Student* and add all the students
listed [above](#what-are-we-working-towards) by clicking on the link **Add A Student** of your
*HasOneComplexTableField* table.

After having added all the students, you will see that, in the tab panel *Student* of all the *Project* pages, the
*HasOneComplexTableField* tables have the same content.
For each *Project* page, you can now affect **one and only one** student to it ( see the
[list](#What_are_we_working_towards?) ).

You will also notice, that you have the possibility to **unselect** a student which will make your *Project* page
without any student affected to it.
**At the moment, the *HasOneComplexTableField* table doesn't manage totally the *1-to-1* relation because you can easily
select the same student for two ( or more ) differents *Project* pages which corresponds to a *1-to-many* relation.**
To use your *HasOneComplexTableField* table for a **1-to-1** relation, make this modification in the class *Project* :
:::php
class Project extends Page {
...
public function getCMSFields() {
...
$tablefield->setParentClass('Project');
$tablefield->setOneToOne();
$fields->addFieldToTab( 'Root.Content.Student', $tablefield );
return $fields;
}
}
Now, you will notice that by checking a student in a *Project* page, you will be unable to select him again in any other
*Project* page which is the definition of a **1-to-1** relation.
## Student - Mentor relation
**A student has one mentor.**
**A mentor has several students.**
This relation is called a **1-to-many** relation.
The first step is to create the mentor object and set the relation with the *Student* data object.
*tutorial/code/Mentor.php*
:::php
<?php
class Mentor extends Page {
static $db = array(
'FirstName' => 'Text',
'Lastname' => 'Text',
'Nationality' => 'Text'
);
static $has_many = array(
'Students' => 'Student'
);
public function getCMSFields() {
$fields = parent::getCMSFields();
$fields->addFieldToTab( 'Root.Content', new TextField( 'FirstName' ) );
$fields->addFieldToTab( 'Root.Content', new TextField( 'Lastname' ) );
$fields->addFieldToTab( 'Root.Content', new TextField( 'Nationality' ) );
return $fields;
}
}
class Mentor_Controller extends Page_Controller {}
*tutorial/code/Student.php*
:::php
class Student extends DataObject {
...
static $has_one = array(
'MyMentor' => 'Mentor'
);
}
This code will create a relationship between the *Student* table and the *Mentor* table by storing the id of the
respective *Mentor* in the *Student* table.
The second step is to add the table in the method *getCMSFields* which will allow you to manage the *has_many* relation.
*tutorial/code/Mentor.php*
:::php
class Mentor extends Page {
...
public function getCMSFields() {
$fields = parent::getCMSFields();
...
$tablefield = new HasManyComplexTableField(
$this,
'Students',
'Student',
array(
'FirstName' => 'FirstName',
'Lastname' => '<NAME>',
'Nationality' => 'Nationality'
),
'getCMSFields_forPopup'
);
$tablefield->setAddTitle( 'A Student' );
$fields->addFieldToTab( 'Root.Students', $tablefield );
return $fields;
}
}
class Mentor_Controller extends Page_Controller {}
To know more about the parameters of the *HasManyComplexTableField* constructor, [check](#project_-_student_relation)
those of the *HasOneComplexTableField* constructor.
<div class="tip" markdown='1'>
Don't forget to rebuild the database using *dev/build?flush=1* before you
proceed to the next part of this tutorial.
</div>
Now that we have created our *Mentor* page type, go into the CMS and create one *Mentor* page for each mentor listed
[above](#what-are-we-working-towards) under a simple *Page* named
**Mentors** for instance.

For each *Mentor* page, you can now affect **many** students created previously ( see the
[list](#What_are_we_working_towards?) ) by going in the tab panel *Students*.

You will also notice, that by checking a student in a *Mentor* page, you will be unable to select him again in any other
*Mentor* page which is the definition of a **1-to-many** relation.
As the *HasOneComplexTableField* table, you also have the possibility not to select any student which will make your
*Mentor* page without any student affected to it.
## Project - Module relation
**A project uses several modules.**
**A module is used by several projects.**
This relation is called a **many-to-many** relation.
The first step is to create the module object and set the relation with the *Project* page type.
*tutorial/code/Module.php*
:::php
<?php
class Module extends DataObject {
static $db = array(
'Name' => 'Text'
);
static $belongs_many_many = array(
'Projects' => 'Project'
);
public function getCMSFields_forPopup() {
$fields = new FieldList();
$fields->push( new TextField( 'Name' ) );
return $fields;
}
}
*tutorial/code/Project.php*
:::php
class Project extends Page {
...
static $many_many = array(
'Modules' => 'Module'
);
}
This code will create a relationship between the *Project* table and the *Module* table by storing the ids of the
respective *Project* and *Module* in a another table named **Project_Modules**.
The second step is to add the table in the method *getCMSFields* which will allow you to manage the *many_many*
relation.
:::php
class Project extends Page {
...
public function getCMSFields() {
$fields = parent::getCMSFields();
...
$modulesTablefield = new ManyManyComplexTableField(
$this,
'Modules',
'Module',
array(
'Name' => 'Name'
),
'getCMSFields_forPopup'
);
$modulesTablefield->setAddTitle( 'A Module' );
$fields->addFieldToTab( 'Root.Modules', $modulesTablefield );
return $fields;
}
}
To know more about the parameters of the *ManyManyComplexTableField* constructor,
[check](#project_-_student_relation) those of the *HasOneComplexTableField*
constructor.
<div class="tip" markdown='1'>
Don't forget to rebuild the database using *dev/build?flush=1* before you
proceed to the next part of this tutorial.
</div>
Select now one of the *Project* page, go in the tab panel *Modules* and add all the modules listed
[above](#what-are-we-working-towards) by clicking on the link **Add A
Module** of your *ManyManyComplexTableField* table.

For each *Project* page, you can now affect **many** modules created previously ( see the
[list](#What_are_we_working_towards?) ) by going in the tab panel
*Modules*.

You will also notice, that you are able to select several times a *Module* on different *Project* pages which is the
definition of a **many-to-many** relation.
As the *HasOneComplexTableField* and *HasManyComplexTableField* table, you also have the possibility not to select any
module which will make your *Project* page without any module affected to it.
## Displaying the data on your website
Now that we have created all the *Page* and *DataObject* classes necessary and the relational tables to
manage the [relations](../topics/datamodel#relations) between them, we would like to see these relations on the website.
We will see in this section how to display all these relations but also how to create a template for a *DataObject*.
For every kind of *Page* or *DataObject*, you can access to their relations thanks to the **control** loop.
**1. GSOC Projects**
Let's start with the *ProjectsHolder* page created before. For this template, we are will display the same table than
[above](#what-are-we-working-towards).

*tutorial/templates/Layout/ProjectsHolder.ss*
:::ss
<% include Menu2 %>
<div id="Content" class="typography">
<% if Level(2) %>
<% include BreadCrumbs %>
<% end_if %>
$Content
<table>
<thead>
<tr>
<th>Project</th>
<th>Student</th>
<th>Mentor</th>
<th>Modules</th>
</tr>
</thead>
<tbody>
<% control Children %>
<tr>
<td>$Title</td>
<td>
<% if MyStudent %>
<% control MyStudent %>
$FirstName $Lastname
<% end_control %>
<% else %>
No Student
<% end_if %>
</td>
<td>
<% if MyStudent %>
<% control MyStudent %>
<% if MyMentor %>
<% control MyMentor %>
$FirstName $Lastname
<% end_control %>
<% else %>
No Mentor
<% end_if %>
<% end_control %>
<% else %>
No Mentor
<% end_if %>
</td>
<td>
<% if Modules %>
<% control Modules %>
$Name
<% end_control %>
<% else %>
No Modules
<% end_if %>
</td>
</tr>
<% end_control %>
</tbody>
</table>
$Form
</div>
<div class="notice" markdown='1'>
If you are using the blackcandy template: You might want to move the `<% include Sidebar %>`
(tutorial/templates/Includes/SideBar.ss) include in the *tutorial/templates/Layout/Page.ss* template above
the typography div to get rid of the bullets
</div>
**2. Project**
We know now how to easily access and show [relations](../topics/datamodel#relations) between *DataObject* in a template.
We can now do the same for every *Project* page by creating its own template.

*tutorial/templates/Layout/Project.ss*
:::ss
<% include Menu2 %>
<div id="Content" class="typography">
<% if Level(2) %>
<% include BreadCrumbs %>
<% end_if %>
$Content
<% if MyStudent %>
<% control MyStudent %>
<p>First Name: <strong>$FirstName</strong></p>
<p>Lastname: <strong>$Lastname</strong></p>
<p>Nationality: <strong>$Nationality</strong></p>
<h3>Mentor</h3>
<% if MyMentor %>
<% control MyMentor %>
<p>First Name: <strong>$FirstName</strong></p>
<p>Lastname: <strong>$Lastname</strong></p>
<p>Nationality: <strong>$Nationality</strong></p>
<% end_control %>
<% else %>
<p>This student doesn't have any mentor.</p>
<% end_if %>
<% end_control %>
<% else %>
<p>There is no any student working on this project.</p>
<% end_if %>
<h3>Modules</h3>
<% if Modules %>
<ul>
<% control Modules %>
<li>$Name</li>
<% end_control %>
</ul>
<% else %>
<p>This project has not used any modules.</p>
<% end_if %>
$Form
</div>
What we would like now is to create a special template for the *DataObject* *Student* and the *Page* *Mentor* which will
be used when we will call directly the variable in the *Project* template. In our case, we will use the same template
because these two classes have the same fields ( FirstName, Surname and Nationality ).
*tutorial/templates/Includes/GSOCPerson.ss*
:::ss
<p>First Name: <strong>$FirstName</strong></p>
<p>Lastname: <strong>$Lastname</strong></p>
<p>Nationality: <strong>$Nationality</strong></p>
Now the template is created, we need to establish the link between the *Student* and *Mentor* classes with their common
template.
To do so, add this code in the two classes. This will create a control on each of those objects which can be called
from templates either within a control block or dot notation.
*tutorial/code/Student.php, tutorial/code/Mentor.php*
:::php
public function PersonalInfo() {
$template = 'GSOCPerson';
return $this->renderWith( $template );
}
We can now modify the *Project.ss* template.
:::ss
...
<% if MyStudent %>
$MyStudent.PersonalInfo
<h3>Mentor</h3>
<% control MyStudent %>
<% if MyMentor %>
$MyMentor.PersonalInfo
<% else %>
<p>This student doesn't have any mentor.</p>
<% end_if %>
<% end_control %>
<% else %>
<p>There is no any student working on this project.</p>
<% end_if %>
...
<div class="notice" markdown='1'>
Remember to add `?flush=1` to the url when refreshing the project page or otherwise you will get a template error
</div>
In the *Project* template, it has been really easy to display the **1-to-1** relation with a *Student* object just by
calling the variable **$MyStudent**. This has been made possible thanks to the code below present in the *Project*
class.
:::php
static $has_one = array(
'MyStudent' => 'Student'
);
However, in the *Student* class, there is no any code relating to the **1-to-1** relation with a *Project* *Page*. So
how to access it from a *Student* *DataObject* ?
**3. Mentor**
In this template, we are gonna try to access the *Project* details from a *Student* *DataObject*.
What we want to do is to access to the *Project* page in the same way than we have done for the other relations
**without modifying the relations between *Page* and *DataObject* and the database structure**.

To do so, we have to create a function in the *Student* class which will return the *Project* linked with it. Let's call
it *MyProject* for instance.
:::php
class Student extends DataObject {
...
public function MyProject() {
return DataObject::get( 'Project', "`MyStudentID` = '{$this->ID}'" );
}
}
We can now use this value in the same way that we have used the other relations.
That's how we can use this function in the *Mentor* template.
*tutorial/templates/Layout/Mentor.ss*
:::ss
<% include Menu2 %>
<div id="Content" class="typography">
<% include BreadCrumbs %>
$Content
<h3>Personal Details</h3>
<p>First Name: <strong>$FirstName</strong></p>
<p>Lastname: <strong>$Lastname</strong></p>
<p>Nationality: <strong>$Nationality</strong></p>
<h3>Students</h3>
<% if Students %>
<table>
<thead>
<tr>
<th>Student</th>
<th>Project</th>
</tr>
</thead>
<tbody>
<% control Students %>
<tr>
<td>$FirstName $Lastname</td>
<td>
<% if MyProject %>
<% control MyProject %>
$Title
<% end_control %>
<% else %>
No Project
<% end_if %>
</td>
</tr>
<% end_control %>
</tbody>
</table>
<% else %>
<p>There is no any student working with this mentor.</p>
<% end_if %>
$Form
</div>
## Summary
This tutorial has demonstrated how easy it is to manage all the type of relations between *DataObject* objects in the
CMS and how to display them on the website.
## Download the code
Download all the [code](http://doc.silverstripe.org/framework/docs/en/tutorials/_images/tutorial5-completecode.zip) for this tutorial.
You can also download the [code](http://doc.silverstripe.org/framework/docs/en/tutorials/_images/tutorial5-completecode-blackcandy.zip) for use in the blackcandy template.
<file_sep>/docs/en/reference/versioned.md
# Versioned
The Versioned class is a `[api:DataObject]` that adds versioning and staging capabilities to the objects.
## Trapping the publication event
Sometimes, you'll want to do something whenever a particular kind of page is published. This example sends an email
whenever a blog entry has been published.
:::php
class Page extends SiteTree {
// ...
public function onAfterPublish() {
mail("<EMAIL>", "Blog published", "The blog has been published");
parent::onAfterPublish();
}
}<file_sep>/docs/en/reference/sitetree.md
# Sitetree
## Introduction
Basic data-object representing all pages within the site tree. The omnipresent *Page* class (located in
*mysite/code/Page.php*) is based on this class.
## Linking
:::php
// wrong
$mylink = $mypage->URLSegment;
// right
$mylink = $mypage->Link(); // alternatively: AbsoluteLink(), RelativeLink()
## Querying
Use *SiteTree::get_by_link()* to correctly retrieve a page by URL, as it taked nested URLs into account (a page URL
might consist of more than one *URLSegment*).
:::php
// wrong
$mypage = DataObject::get_one('SiteTree', '"URLSegment" = \'<mylink>\'');
// right
$mypage = SiteTree::get_by_link('<mylink>');
## Nested/Hierarchical URLs
In a nutshell, the nested URLs feature means that your site URLs now reflect the actual parent/child page structure of
your site. The URLs map directly to the chain of parent and child pages. The
below table shows a quick summary of what these changes mean for your site:
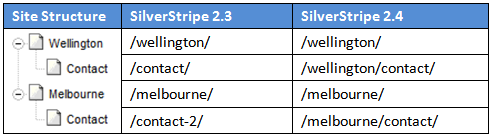
## Limiting Children/Parent
By default, any page type can be the child of any other page type. However, there are 4 static properties that can be
used to set up restrictions that will preserve the integrity of the page hierarchy.
:::php
class BlogHolder extends Page {
// Blog holders can only contain blog entries
static $allowed_children = array("BlogEntry");
static $default_child = "BlogEntry";
...
class BlogEntry extends Page {
// Blog entries can't contain children
static $allowed_children = "none";
static $default_parent = "blog";
static $can_be_root = false;
...
class Page extends SiteTree {
// Don't let BlogEntry pages be underneath Pages. Only underneath Blog holders.
static $allowed_children = array("*Page,", "BlogHolder");
}
* **allowed_children:** This can be an array of allowed child classes, or the string "none" - indicating that this page
type can't have children. If a classname is prefixed by "*", such as "*Page", then only that class is allowed - no
subclasses. Otherwise, the class and all its subclasses are allowed.
* **default_child:** If a page is allowed more than 1 type of child, you can set a default. This is the value that
will be automatically selected in the page type dropdown when you create a page in the CMS.
* **default_parent:** This should be set to the *URLSegment* of a specific page, not to a class name. If you have
asked to create a page of a particular type that's not allowed underneath the page that you have selected, then the
default_parent page will be selected. For example, if you have a gallery page open in the CMS, and you select add blog
entry, you can set your site up to automatically select the blog page as a parent.
* **can_be_root:** This is a boolean variable. It lets you specify whether the given page type can be in the top
level.
Note that there is no allowed_parents control. To set this, you will need to specify the allowed_children of all other
page types to exclude the page type in question. IMO this is less than ideal; it's possible that in a future release we
will add allowed_parents, but right now we're trying to limit the amount of mucking around with the API we do.
Here is an overview of everything you can add to a class that extends sitetree. NOTE: this example will not work, but
it is a good starting point, for choosing your customisation.
:::php
class Page extends SiteTree {
// tree customisation
static $icon = "";
static $allowed_children = array("SiteTree"); // set to string "none" or array of classname(s)
static $default_child = "Page"; //one classname
static $default_parent = null; // NOTE: has to be a URL segment NOT a class name
static $can_be_root = true; //
static $hide_ancestor = null; //dont show ancestry class
// extensions and functionality
static $versioning = array();
static $default_sort = "Sort";
/static $extensions = array();
public static $breadcrumbs_delimiter = " » ";
public function canCreate() {
//here is a trick to only allow one (e.g. holder) of a page
return !DataObject::get_one($this->class);
}
public function canDelete() {
return false;
}
public function getCMSFields() {
$fields = parent::getCMSFields();
return $fields;
}
## Recipes
### Automatic Child Selection
By default, `[api:SiteTree]` class to build a tree using the ParentID field. However, sometimes, you want to change
this default behaviour.
For example, in our e-commerce module, we use a many-to-many join, Product::Parents, to let you put Products in multiple
groups. Here's how to implement such a change:
* **Set up your new data model:** Create the appropriate many-many join or whatever it is that you're going to use to
store parents.
* **Define stageChildren method:** This method should return the children of the current page, for the current version.
If you use DataObject::get, the `[api:Versioned]` class will rewrite your query to access the live site when
appropriate.
* **Define liveChildren method:** The method should return the children of the current page, for the live site.
Both the CMS and the site's data controls will make use of this, so navigation, breadcrumbs, etc will be updated. If 1
node appears in the tree more than once, it will be represented differently.
**TO DO:** Work out this representation.
### Custom Children Getters
Returning custom children for a specific `SiteTree` subclass can be handy to influence the tree display within the
CMS. An example of custom children might be products which belong to multiple categories. One category would get its
products from a `$many_many` join rather than the default relations.
Children objects are generated from two functions `stageChildren()` and `liveChildren()` and the tree generation in
the CMS is calculated from `numChildren()`. Please keep in mind that the returned children should still be instances
of `SiteTree`.
Example:
:::php
class MyProduct extends Page {
static $belongs_many_many = array(
'MyCategories' => 'MyCategory'
);
}
class MyCategory extends Page {
static $many_many = array(
'MyProducts' => 'MyProduct'
);
public function stageChildren($showAll = false) {
// @todo Implement $showAll
return $this->MyProducts();
}
public function liveChildren($showAll = false) {
// @todo Implement $showAll
return $this->MyProducts();
}
public function numChildren() {
return $this->MyProducts()->Count();
}
} }
}
### Multiple parents in the tree
The `[api:LeftAndMain]` tree supports multiple parents. We overload CMSTreeClasses and make it include "manyparents" in
the class list.
:::php
public function CMSTreeClasses($controller) {
return parent::CMSTreeClasses($controller) . ' manyparents';
}
Don't forget to define a new Parent() method that also references your new many-many join (or however it is you've set
up the hierarchy!
:::php
public function getParent() {
return $this->Parent();
}
public function Parent() {
$parents = $this->Parents();
if($parents) return $parents->First();
}
Sometimes, you don't want to mess with the CMS in this manner. In this case, leave stageChildren() and liveChildren()
as-is, and instead make another method, such as ChildProducts(), to get the data from your many-many join.
### Dynamic Grouping
Something that has been talked about [here](http://www.silverstripe.com/site-builders-forum/flat/15416#post15940) is the
concept of "dynamic grouping". In essence, it means adding navigational tree nodes to the tree that don't correspond to
a database record.
How would we do this? In our example, we're going to update BlogHolder to show BlogEntry children grouped into months.
We will create a class called BlogMonthTreeNode, which will extend ViewableData instead of DataRecord, since it's not
saved into the database. This will represent our dynamic groups.
### LeftAndMain::getSiteTreeFor()
Currently LeftAndMain::getSiteTreeFor() Calls LeftAndMain::getRecord($id) to get a new record. We need to instead
create a new public function getTreeRecord($id) which will be able to create BlogMonthTreeNode objects as well as look up
SiteTree records from the database.
The IDs don't **need** be numeric; so we can set the system to allow for 2 $id formats.
* (ID): A regular SiteTree object
* BlogMonthTreeNode-(BlogHolderID)-(Year)-(Month): A BlogMonthTreeNode object
To keep the code generic, we will assume that if the $id isn't numeric, then we should explode('-', $id), and use the
first part as the classname, and all the remaining parts as arguments to the constructor.
Your BlogMonthTreeNode constructor will then need to take $blogHolderID, $year, $month as arguments.
### Divorcing front-end site's Children() and the CMS's AllChildrenIncludingDeleted()
We need a way of cleanly specifying that there are two different child sources - children for the CMS tree, and children
for the front-end site.
* We currently have stageChildren() / liveChildren()
* We should probably add cmsStageChildren() and cmsLiveChildren() into the mix, for SiteTree.
AllChildrenIncludingDeleted() could then call the "cms..." versions of the functions, but if we were to to this, we
should probably rename AllChildrenIncludingDeleted() to CMSTreeChildren() or something like that.
### BlogHolder::cmsStageChildren() & BlogHolder::cmsLiveChildren()
We will need to define these methods, to
* Get the stage/live children of the page, grouped by month
* For each entry returned, generate a new BlogMonthTreeNode object.
* Return that as a dataobjectset.
### BlogMonthTreeNode
* Parameter 'ID': should return 'BlogMonthTreeNode-(BlogHolderID)-(Year)-(Month)'. You can do this by implementing
getID().
* Methods cmsStageChildren() and cmsLiveChildren(): These should return the blog-entries for that month.
After that, there will be some other things to tweak, like the tree icons.
### Where to from here?
This is a lot of work for the specific example of blog-entries grouped by month. Instead of BlogMonthTreeNode, you
could genericise this to a DynamicTreeGroup class, which would let you specify the parent node, the type of grouping,
and the specific group.
## TODO
Clean up this documentation
## API Documentation
`[api:Sitetree]`
<file_sep>/docs/en/topics/testing/testing-guide-troubleshooting.md
# Troubleshooting
Part of the [SilverStripe Testing Guide](testing-guide).
## I can't run my new test class
If you've just added a test class, but you can't see it via the web interface, chances are, you haven't flushed your
manifest cache - append `?flush=1` to the end of your URL querystring.
## Class 'PHPUnit_Framework_MockObject_Generator' not found
This is due to an upgrade in PHPUnit 3.5 which PEAR doesn't handle correctly.<br>
It can be fixed by running the following commands:
pear install -f phpunit/DbUnit
pear install -f phpunit/PHPUnit_MockObject
pear install -f phpunit/PHPUnit_Selenium
<file_sep>/forms/gridfield/GridFieldLevelup.php
<?php
class GridFieldLevelup implements GridField_HTMLProvider{
/**
* @var integer - the record id of the level up to
*/
protected $levelID = null;
/**
*
* @param integer $levelID - the record id of the level up to
*/
public function __construct($levelID = null) {
if($levelID && is_numeric($levelID)) {
$this->levelID = $levelID;
}
}
public function getHTMLFragments($gridField) {
$modelClass = $gridField->getModelClass();
if(isset($_GET['ParentID']) && $_GET['ParentID']){
$modelObj = DataObject::get_by_id($modelClass, $_GET['ParentID']);
if(is_callable(array($modelObj, 'getParent'))){
$levelup = $modelObj->getParent();
if(!$levelup){
$parentID = 0;
}else{
$parentID = $levelup->ID;
}
}
//$controller = $gridField->getForm()->Controller();
$forTemplate = new ArrayData(array(
'UpLink' => sprintf(
'<a class="cms-panel-link list-parent-link" href="?ParentID=%d&view=list" data-target-panel="#Form_ListViewForm" data-pjax="ListViewForm">%s</a>',
$parentID,
_t('GridField.LEVELUP', 'Level up' )
),
));
return array(
'before' => $forTemplate->renderWith('GridFieldLevelup'),
//'header' => $forTemplate->renderWith('GridFieldLevelup_Row'),
);
}
}
}
?><file_sep>/docs/en/reference/sqlquery.md
# SQL Query
## Introduction
An object representing a SQL query. It is easier to deal with object-wrappers than string-parsing a raw SQL-query. This
object is used by `[api:DataObject]`, though...
A word of caution: Dealing with low-level SQL is not encouraged in the SilverStripe [datamodel](/topics/datamodel) for various
reasons. You'll break the behaviour of:
* Custom getters/setters
* DataObject::onBeforeWrite/onBeforeDelete
* Automatic casting
* Default-setting through object-model
* `[api:DataObject]`
* Database abstraction
We'll explain some ways to use *SELECT* with the full power of SQL, but still maintain a connection to the SilverStripe
[datamodel](/topics/datamodel).
## Usage
### SELECT
:::php
$sqlQuery = new SQLQuery();
$sqlQuery->select = array(
'Firstname AS Name',
'YEAR(Birthday) AS BirthYear'
);
$sqlQuery->from = "
Player
LEFT JOIN Team ON Player.TeamID = Team.ID
";
$sqlQuery->where = "
YEAR(Birthday) = 1982
";
// $sqlQuery->orderby = "";
// $sqlQuery->groupby = "";
// $sqlQuery->having = "";
// $sqlQuery->limit = "";
// $sqlQuery->distinct = true;
// get the raw SQL
$rawSQL = $sqlQuery->sql();
// execute and return a Query-object
$result = $sqlQuery->execute();
### DELETE
:::php
// ...
$sqlQuery->delete = true;
### INSERT/UPDATE
(currently not supported -see below for alternative solutions)
## Working with results
The result is an array lightly wrapped in a database-specific subclass of `[api:Query]`. This class implements the
*Iterator*-interface defined in PHP5, and provides convenience-methods for accessing the data.
### Iterating
:::php
foreach($result as $row) {
echo $row['BirthYear'];
}
### Quick value checking
Raw SQL is handy for performance-optimized calls.
:::php
class Team extends DataObject {
public function getPlayerCount() {
$sqlQuery = new SQLQuery(
"COUNT(Player.ID)",
"Team LEFT JOIN Player ON Team.ID = Player.TeamID"
);
return $sqlQuery->execute()->value();
}
Way faster than dealing with `[api:DataObject]`s, but watch out for premature optimisation:
:::php
$players = $myTeam->Players();
echo $players->Count();
### Mapping
Useful for creating dropdowns.
:::php
$sqlQuery = new SQLQuery(
array('YEAR(Birthdate)', 'Birthdate'),
'Player'
);
$map = $sqlQuery->execute()->map();
$field = new DropdownField('Birthdates', 'Birthdates', $map);
### "Raw" SQL with DB::query()
This is not recommended for most cases, but you can also use the SilverStripe database-layer to fire off a raw query:
:::php
DB::query("UPDATE Player SET Status='Active'");
One example for using a raw DB::query is when you are wanting to order twice in the database:
:::php
$records = DB::query('SELECT *, CASE WHEN "ThumbnailID" = 0 THEN 2 ELSE 1 END AS "HasThumbnail" FROM "TempDoc" ORDER BY "HasThumbnail", "Name" ASC');
$items = singleton('TempDoc')->buildDataObjectSet($records);
This CASE SQL creates a second field "HasThumbnail" depending if "ThumbnailID" exists in the database which you can then
order by "HasThumbnail" to make sure the thumbnails are at the top of the list and then order by another field "Name"
separately for both the items that have a thumbnail and then for those that don't have thumbnails.
### "Semi-raw" SQL with buildSQL()
You can gain some ground on the datamodel-side when involving the selected class for querying. You don't necessarily
need to call *buildSQL* from a specific object-instance, a *singleton* will do just fine.
:::php
$sqlQuery = singleton('Player')->buildSQL(
'YEAR(Birthdate) = 1982'
);
This form of building a query has the following advantages:
* Respects DataObject::$default_sort
* Automatically LEFT JOIN on all base-tables (see [database-structure](database-structure))
* Selection of *ID*, *ClassName*, *RecordClassName*, which are necessary to use *buildDataObjectSet* later on
* Filtering records for correct *ClassName*
### Transforming a result to `[api:DataObjectSet]`
This is a commonly used technique inside SilverStripe: Use raw SQL, but transfer the resulting rows back into
`[api:DataObject]`s.
:::php
$sqlQuery = new SQLQuery();
$sqlQuery->select = array(
'Firstname AS Name',
'YEAR(Birthday) AS BirthYear',
// IMPORTANT: Needs to be set after other selects to avoid overlays
'Player.ClassName AS ClassName',
'Player.ClassName AS RecordClassName',
'Player.ID AS ID'
);
$sqlQuery->from = array(
"Player",
"LEFT JOIN Team ON Player.TeamID = Team.ID"
);
$sqlQuery->where = array(
"YEAR(Player.Birthday) = 1982"
);
$result = $sqlQuery->execute();
var_dump($result->first()); // array
// let Silverstripe work the magic
$myDataObjectSet = singleton('Player')->buildDataObjectSet($result);
var_dump($myDataObjectSet->First()); // DataObject
// this is where it gets tricky
$myFirstPlayer = $myDataObjectSet->First();
var_dump($myFirstPlayer->Name); // 'John'
var_dump($myFirstPlayer->Firstname); // undefined, as it was not part of the SELECT-clause;
var_dump($myFirstPlayer->Surname); // undefined, as it was not part of the SELECT-clause
// lets assume that class Player extends BasePlayer,
// and BasePlayer has a database-column "Status"
var_dump($myFirstPlayer->Status); // undefined, as we didn't LEFT JOIN the BasePlayer-table
**CAUTION:** Depending on the selected columns in your query, you might get into one of the following scenarios:
* Not all object-properties accessible: You need to take care of selecting the right stuff yourself
* Overlayed object-properties: If you *LEFT JOIN* a table which also has a column 'Birthdate' and do a global select on
this table, you might not be able to access original object-properties.
* You can't create `[api:DataObject]`s where no scalar record-data is available, e.g. when using *GROUP BY*
* Naming conflicts with custom getters: A getter like Player->getName() will overlay the column-data selected in the
above example
Be careful when saving back `[api:DataObject]`s created through *buildDataObjectSet*, you might get strange side-effects due to
the issues noted above.
## Using FormFields with custom SQL
Some subclasses of `[api:FormField]` for ways to create sophisticated report-tables based on SQL.
## Related
* [datamodel](/topics/datamodel)
* `[api:DataObject]`
* [database-structure](database-structure)
## API Documentation
`[api:SQLQuery]`<file_sep>/view/GenericTemplateGlobalProvider.php
<?php
class GenericTemplateGlobalProvider implements TemplateGlobalProvider {
public static function get_template_global_variables() {
return array(
'ModulePath'
);
}
/**
* @var array Module paths
*/
public static $modules = array(
'framework' => FRAMEWORK_DIR,
'frameworkadmin' => FRAMEWORK_ADMIN_DIR,
'thirdparty' => THIRDPARTY_DIR,
'assets' => ASSETS_DIR
);
/**
* Given some pre-defined modules, return the filesystem path of the module.
* @param string $name Name of module to find path of
* @return string
*/
public static function ModulePath($name) {
if(isset(self::$modules[$name])) {
return self::$modules[$name];
} else {
throw new InvalidArgumentException(sprintf('%s is not a supported argument. Possible values: %s', $name, implode(', ', self::$modules)));
}
}
}
<file_sep>/model/GroupedList.php
<?php
/**
* A list decorator that allows a list to be grouped into sub-lists by common
* values of a field.
*
* @package framework
* @subpackage model
*/
class GroupedList extends SS_ListDecorator {
/**
* @param string $index
* @return ArrayList
*/
public function groupBy($index) {
$result = array();
foreach ($this->list as $item) {
$key = is_object($item) ? $item->$index : $item[$index];
if (array_key_exists($key, $result)) {
$result[$key]->push($item);
} else {
$result[$key] = new ArrayList(array($item));
}
}
return $result;
}
/**
* @param string $index
* @param string $children
* @return ArrayList
*/
public function GroupedBy($index, $children = 'Children') {
$grouped = $this->groupBy($index);
$result = new ArrayList();
foreach ($grouped as $indVal => $list) {
$result->push(new ArrayData(array(
$index => $indVal,
$children => $list
)));
}
return $result;
}
}
<file_sep>/docs/en/reference/built-in-page-controls.md
# Built-in Page Controls
Ever wonder when you use `$Title` and `<% Control Children %>` what else you can call in the templates?. This page is
here to help with a guide on what template controls you can call.
**Note for advanced users:** These built-in page controls are defined in the [api:SiteTree] classes, which are the
'root' data-object and controller classes for all the sites. So if you're dealing with something that isn't a sub-class
of one of these, our handy reference to 'built-in page controls' won't be so relevant.
## Page controls that can't be nested
These page controls are defined on the **controller** which means they can only be used at a top level, not nested
within another page control.
### Controlling Menus Datafeeds
#### <% control Menu(1) %>, <% control Menu(2) %>, ...
Returns a fixed level menu. Because this only works in the top level, you can't use it for nested menus. Use
`<% control Children %>` instead. You can nest `<% control Children %>`.
#### <% control ChildrenOf(page-url) %>
This will create a datafeed of the children of the given page. Handy if you want a list of the subpages under staff (eg
the staff) on the homepage etc
### Controlling Certain Pages
#### <% control Level(1) %>, <% control Level(2) %>, $Level(1).Title, $Level(2).Content, etc
Returns the current section of the site that we're in, at the level specified by the numbers. For example, imagine
you're on the page __about us > staff > <NAME>__:
* `<% control Level(1) %>` would return the about us page
* `<% control Level(2) %>` would return the staff page
* `<% control Level(3) %>` would return the bob marley page
#### <% control Page(my-page) %>$Title<% end_control %>
"Page" will return a single page from the site tree, looking it up by URL. You can use it in the `<% control %>` format.
Can't be called using `$Page(my-page).Title`.
## Page controls that can be used anywhere
These are defined in the data-object and so can be used as nested page controls. Lucky us! we can control Children of
Children of Children for example.
### Conditional Logic
SilverStripe supports a simple set of conditional logic
:::ss
<% if Foo %>
// if Foo is true or an object do this
<% else_if Bar %>
// if Bar is true or an object do this
<% else %>
// then do this by default
<% end_if %>
See more information on conditional logic on [templates](/topics/templates).
### Site wide settings
Since 2.4.0, SilverStripe provides a generic interface for accessing global properties such as *Site name* or *Site tag
line*. This interface is implemented by the [api:SiteConfig] class.
### Controlling Parents and Children
#### <% control Children %>
This will return the children of the current page as a nested datafeed. Useful for nested navigations such as pop-out
menus.
#### <% control AllChildren %>
This will show all children of a page even if the option 'show in menus?' is unchecked in the tab panel behaviour.
#### <% control Parent %> or $Parent.Title, $Parent.Content, etc
This will return the parent page. The $ variable format lets us reference an attribute of the parent page directly.
### Site Navigation - Breadcrumbs
#### $Breadcrumbs
This will return a breadcrumbs widget for the current page. You can call this on any SiteTree descendant, so, for
example, you could display the breadcrumbs of every search result if you wanted. The Breadcrumbs method returns a string
of text, so this can't be used as a control block (that is, you can't usefully say "<% control Breadcrumbs %>"). You can
limit the number of items in the breadcrumbs, as well as whether the breadcrumb items are links.
#### $Breadcrumbs(3)
This returns a maximum of 3 pages in the breadcrumb list, which can be handy if you want to limit the size of your
breadcrumbs to conform to your page design.
#### <% control Breadcrumbs(3, true) %>
This returns the same, but without any links. This is handy if you want to put the breadcrumb list into another link
tag.
### Links and Classes
#### $LinkingMode, $LinkOrCurrent and $LinkOrSection
These return different linking modes. $LinkingMode provides the greatest control, outputting 3 different strings:
* link: Neither this page nor any of its children are current open.
* section: A child of this page is currently open, which means that we're currently in this section of the site.
* current: This page is currently open.
A useful way of using this is in your menus. You can use the following code below to generate class="current" or
class="section" on your links. Take the following code
:::ss
<li><a href="$Link" class="$LinkingMode">$Title</a></li>
When viewed on the Home page it will render like this
:::ss
<li><a href="home/" class="current">Home</a></li>
`$LinkOrCurrent` ignores the section status, returning link instead. `$LinkOrSection` ignores the current status, returning section instead. Both of these options can simplify your CSS when you only have 2 different cases to consider.
#### <% if LinkOrCurrent = current %>
This is an alternative way to set up your menus - if you want different HTML for the current menu item, you can do
something like this:
:::ss
<% if LinkOrCurrent = current %>
<strong>$Title</strong>
<% else %>
<a href="$Link">$Title</a>
<% end_if %>
#### <% if LinkOrSection = section %>
Will return true if you are on the current page OR a child page of the page. Useful for menus which you only want to
show a second level menu when you are on that page or a child of it
#### <% if InSection(page-url) %>
This if block will pass if we're currently on the page-url page or one of its children.
### Titles and CMS Defined Options
#### $MetaTags
This returns a segment of HTML appropriate for putting into the `<head>` tag. It will set up title, keywords and
description meta-tags, based on the CMS content. If you don't want to include the title-tag (for custom templating), use
**$MetaTags(false)**.
#### $MenuTitle
This is the title that you should put into navigation menus. CMS authors can choose to put a different menu title from
the main page title.
#### $Title
This is the title of the page which displays in the browser window and usually is the title of the page.
:::ss
<h1>$Title</h1>
#### $URLSegment
This returns the part of the URL of the page you're currently on. Could be handy to use as an id on your body-tag. (
when doing this, watch out that it doesn't create invalid id-attributes though.). This is useful for adding a class to
the body so you can target certain pages. Watch out for pages named clear or anything you might have used in your CSS
file
:::ss
<body class="$URLSegment">
#### $ClassName
Returns the ClassName of the PHP object. Eg if you have a custom HomePage page type with `$ClassName` in the template, it
will return "HomePage"
#### $BaseHref
Returns the base URL for the current site. This is used to populate the `<base>` tag by default, so if you want to
override `<% base_tag %>` with a specific piece of HTML, you can do something like `<base href="$BaseHref"></base>`
### Controlling Members and Visitors Data
#### <% control CurrentMember %>, <% if CurrentMember %> or $CurrentMember.FirstName
CurrentMember returns the currently logged in member, if there is one. All of their details or any special Member page
controls can be called on this. Alternately, you can use `<% if CurrentMember %>` to detect whether someone has logged
in. To Display a welcome message you can do
:::ss
<% if CurrentMember %>
Welcome Back, $CurrentMember.FirstName
<% end_if %>
If the user is logged in this will print out
:::ss
Welcome Back, Admin
#### <% if PastMember %>
Detect the visitor's previous experience with the site. `$PastMember` will return true if the visitor has signed up or
logged in on the site before.
Note that as of version 2.4 `$PastVisitor` is deprecated. If you wish to check if a visitor has been to the site before,
set a cookie with `Cookie::set()` and test for it with `Cookie::get()`.
### Date and Time
#### $Now.Nice, $Now.Year
`$Now` returns the current date. You can call any of the methods from the [api:Date] class on
it.
#### $Created.Nice, $Created.Ago
`$Created` returns the time the page was created, `$Created.Ago` returns how long ago the page was created. You can also
call any of methods of the [api:Date] class on it.
#### $LastEdited.Nice, $LastEdited.Ago
`$LastEdited `returns the time the page was modified, `$LastEdited.Ago` returns how long ago the page was modified. You
can also call any of methods of the [api:Date] class on it.
### DataObjectSet Options
If you are using a DataObjectSet you have a wide range of methods you can call on it from the templates
#### <% if Even %>, <% if Odd %>, $EvenOdd
These controls can be used to do zebra-striping. `$EvenOdd` will return 'even' or 'odd' as appropriate.
#### <% if First %>, <% if Last %>, <% if Middle %>, $FirstLast
These controls can be used to set up special behaviour for the first and last records of a datafeed. `<% if Middle %>` is
set when neither first not last are set. `$FirstLast` will be 'first', 'last', or ''.
#### $Pos, $TotalItems
`$TotalItems` will return the number of items on this page of the datafeed, and `$Pos` will return a counter starting at 1.
#### $Top
When you're inside a control loop in your template, and want to reference methods on the current controller you're on,
breaking out of the loop to get it, you can use `$Top` to do so. For example:
:::ss
$URLSegment
<% control News %>
$URLSegment <!-- may not return anything, as you're requesting URLSegment on the News objects -->
$Top.URLSegment <!-- returns the same as $URLSegment above -->
<% end_control %>
## Properties of a datafeed itself, rather than one of its items
If we have a control such as `<% control SearchResults %>`, there are some properties, such as `$SearchResults.NextLink`,
that aren't accessible within `<% control SearchResults %>`. These can be used on any datafeed.
### Search Results
#### <% if SearchResults.MoreThanOnePage %>
Returns true when we have a multi-page datafeed, restricted with a limit.
#### $SearchResults.NextLink, $SearchResults.PrevLink
This returns links to the next and previous page in a multi-page datafeed. They will return blank if there's no
appropriate page to go to, so `$PrevLink` will return blank when you're on the first page. You can therefore use
`<% if PrevLink %>` to keep your template tidy.
#### $SearchResults.CurrentPage, $SearchResults.TotalPages
CurrentPage returns the number of the page you're currently on, and TotalPages returns the total number of pages.
#### $SearchResults.TotalItems
This returns the total number of items across all pages.
#### <% control SearchResults.First %>, <% control SearchResults.Last %>
These controls return the first and last item on the current page of the datafeed.
#### <% control SearchResults.Pages %>
This will return another datafeed, listing all of the pages in this datafeed. It will have the following data
available:
* **$PageNum:** page number, starting at 1
* **$Link:** a link straight to that page
* `<% if CurrentBool %>`:** returns true if you're currently on that page
`<% control SearchResults.Pages(30) %>` will show a maximum of 30 pages, useful in situations where you could get 100s of
pages returned.
#### $SearchResults.UL
This is a quick way of generating a `<ul>` containing an `<li>` and `<a>` for each item in the datafeed. Usually too
restricted to use in a final application, but handy for debugging stuff.
## Quick Reference
Below is a list of fields and methods that are typically available for templates (grouped by their source) - use this as
a quick reference (not all of them are described above):
### All methods available in Page_Controller
$NexPageLink, $Link, $RelativeLink, $ChildrenOf, $Page, $Level, $Menu, $Section2, $LoginForm, $SilverStripeNavigator,
$PageComments, $Now, $LinkTo, $AbsoluteLink, $CurrentMember, $PastVisitor, $PastMember, $XML_val, $RAW_val, $SQL_val,
$JS_val, $ATT_val, $First, $Last, $FirstLast, $MiddleString, $Middle, $Even, $Odd, $EvenOdd, $Pos, $TotalItems,
$BaseHref, $Debug, $Top
### All fields available in Page_Controller
$ID, $ClassName, $Created, $LastEdited, $URLSegment, $Title, $MenuTitle, $Content, $MetaTitle, $MetaDescription,
$MetaKeywords, $ShowInMenus, $ShowInSearch, $HomepageForDomain, $ProvideComments, $Sort, $LegacyURL, $HasBrokenFile,
$HasBrokenLink, $Status, $ReportClass, $ParentID, $Version, $EmailTo, $EmailOnSubmit, $SubmitButtonText,
$OnCompleteMessage, $Subscribe, $AllNewsletters, $Subject, $ErrorCode, $LinkedPageID, $RedirectionType, $ExternalURL,
$LinkToID, $VersionID, $CopyContentFromID, $RecordClassName
### All methods available in Page
$Link, $LinkOrCurrent, $LinkOrSection, $LinkingMode, $ElementName, $InSection, $Comments, $Breadcrumbs, $NestedTitle,
$MetaTags, $ContentSource, $MultipleParents, $TreeTitle, $CMSTreeClasses, $Now, $LinkTo, $AbsoluteLink, $CurrentMember,
$PastVisitor, $PastMember, $XML_val, $RAW_val, $SQL_val, $JS_val, $ATT_val, $First, $Last, $FirstLast, $MiddleString,
$Middle, $Even, $Odd, $EvenOdd, $Pos, $TotalItems, $BaseHref, $Top
### All fields available in Page
$ID, $ClassName, $Created, $LastEdited, $URLSegment, $Title, $MenuTitle, $Content, $MetaTitle, $MetaDescription,
$MetaKeywords, $ShowInMenus, $ShowInSearch, $HomepageForDomain, $ProvideComments, $Sort, $LegacyURL, $HasBrokenFile,
$HasBrokenLink, $Status, $ReportClass, $ParentID, $Version, $EmailTo, $EmailOnSubmit, $SubmitButtonText,
$OnCompleteMessage, $Subscribe, $AllNewsletters, $Subject, $ErrorCode, $LinkedPageID, $RedirectionType, $ExternalURL,
$LinkToID, $VersionID, $CopyContentFromID, $RecordClassName
<file_sep>/docs/en/reference/dataobject.md
# DataObject
## Introduction
A single database record & abstract class for the data-access-model.
## Usage
* [datamodel](/topics/datamodel): The basic pricinples
* [data-types](/topics/data-types): Casting and special property-parsing
* `[api:DataObject]`: A "container" for DataObjects
## Basics
The call to `DataObject->getCMSFields()` is the centerpiece of every data administration interface in SilverStripe,
which returns a `[api:FieldList]`''.
:::php
class MyPage extends Page {
public function getCMSFields() {
$fields = parent::getCMSFields();
$fields->addFieldToTab('Root.Content',new CheckboxField('CustomProperty'));
return $fields;
}
}
## Scaffolding Formfields
These calls retrieve a `[api:FieldList]` for the area where you intend to work with the scaffolded form.
### For the CMS
:::php
$fields = singleton('MyDataObject')->getCMSFields();
### For the Frontend
Used for simple frontend forms without relation editing or `[api:TabSet] behaviour. Uses `scaffoldFormFields()` by
default. To customize, either overload this method in your subclass, or extend it by `DataExtension->updateFormFields()`.
:::php
$fields = singleton('MyDataObject')->getFrontEndFields();
## Customizing Scaffolded Fields
This section covers how to enhance the default scaffolded form fields from above. It is particularly useful when used
in conjunction with the `[api:ModelAdmin]` in the CMS to make relevant data administration interfaces.
### Searchable Fields
The `$searchable_fields` property uses a mixed array format that can be used to further customize your generated admin
system. The default is a set of array values listing the fields.
Example: Getting predefined searchable fields
:::php
$fields = singleton('MyDataObject')->searchableFields();
Example: Simple Definition
:::php
class MyDataObject extends DataObject {
static $searchable_fields = array(
'Name',
'ProductCode'
);
}
Searchable fields will be appear in the search interface with a default form field (usually a `[api:TextField]`) and a default
search filter assigned (usually an `[api:ExactMatchFilter]`). To override these defaults, you can specify additional information
on `$searchable_fields`:
:::php
class MyDataObject extends DataObject {
static $searchable_fields = array(
'Name' => 'PartialMatchFilter',
'ProductCode' => 'NumericField'
);
}
If you assign a single string value, you can set it to be either a `[api:FormField]` or `[api:SearchFilter]`. To specify both, you can
assign an array:
:::php
class MyDataObject extends DataObject {
static $searchable_fields = array(
'Name' => array(
'field' => 'TextField',
'filter' => 'PartialMatchFilter',
),
'ProductCode' => array(
'title' => 'Product code #',
'field' => 'NumericField',
'filter' => 'PartialMatchFilter',
),
);
}
To include relations (''$has_one'', `$has_many` and `$many_many`) in your search, you can use a dot-notation.
:::php
class Team extends DataObject {
static $db = array(
'Title' => 'Varchar'
);
static $many_many = array(
'Players' => 'Player'
);
static $searchable_fields = array(
'Title',
'Players.Name',
);
}
class Player extends DataObject {
static $db = array(
'Name' => 'Varchar',
'Birthday' => 'Date'
);
static $belongs_many_many = array(
'Teams' => 'Team'
);
}
### Summary Fields
Summary fields can be used to show a quick overview of the data for a specific `[api:DataObject]` record. Most common use is
their display as table columns, e.g. in the search results of a `[api:ModelAdmin]` CMS interface.
Example: Getting predefined summary fields
:::php
$fields = singleton('MyDataObject')->summaryFields();
Example: Simple Definition
:::php
class MyDataObject extends DataObject {
static $db = array(
'Name' => 'Text',
'OtherProperty' => 'Text',
'ProductCode' => 'Int',
);
static $summary_fields = array(
'Name',
'ProductCode'
);
}
To include relations in your summaries, you can use a dot-notation.
:::php
class OtherObject extends DataObject {
static $db = array(
'Title' => 'Varchar'
);
}
class MyDataObject extends DataObject {
static $db = array(
'Name' => 'Text'
);
static $has_one = array(
'OtherObject' => 'OtherObject'
);
static $summary_fields = array(
'Name',
'OtherObject.Title'
);
}
## API Documentation
`[api:DataObject]`
<file_sep>/docs/en/misc/subversion.md
## Subversion
Subversion [website](http://subversion.tigris.org) is a **version control system**.
You can browse our Subversion "code repository" [here](http://open.silverstripe.com/browser), however we now use GIT to
manage our modules (see [Contributing](contributing)).
This page only describes SilverStripe-specific information on how to handle subversion. For a general introduction,
please read the [Official Subversion Book](http://svnbook.red-bean.com/) (available free online).
Start with the ["Basic Usage" chapter](http://svnbook.red-bean.com/en/1.5/svn.tour.html).
### Clients
Make sure you have an updated [subversion client](http://subversion.tigris.org/links.html#clients) installed.
Subversion 1.5 or higher is required for features such as relative externals and merge tracking.
### Checkout / Download
See [Download SilverStripe](http://silverstripe.org/download) and the
["Update your working copy" chapter](http://svnbook.red-bean.com/en/1.5/svn.tour.cycle.html#svn.tour.cycle.update).
### Committing
The SilverStripe core and modules require permission to commit code.
Please have a look at our [contributors guidelines](contributing) to find out how you can gain access.
### Configuring subversion for correct newline handling
You should configure your subversion client to set the ''svn:eol-style'' property to ''native'' on all text files checked into the system. This will ensure that we don't run into troubles, getting non-unix newlines inside our repository.
To do this, edit your ''~/.subversion/config'' file on your development machine, and add the following settings. Note that if you already have ''[miscellany]'' and ''[auto-props]'' sections, you should combine these settings with the existing sections, rather than doing a blind copy & paste to the end of the file.
:::php
[miscellany]
enable-auto-props = yes
// Section for configuring automatic properties.
[auto-props]
*.js = svn:eol-style=native
*.html = svn:eol-style=native
*.inc = svn:eol-style=native
*.css = svn:eol-style=native
*.php = svn:eol-style=native
*.xml = svn:eol-style=native
*.csv = svn:eol-style=native
*.htm = svn:eol-style=native
*.ini = svn:eol-style=native
*.json = svn:eol-style=native
*.php5 = svn:eol-style=native
*.py = svn:eol-style=native
*.ss = svn:eol-style=native
*.yml = svn:eol-style=native
*.yaml = svn:eol-style=native
*.xhtml = svn:eol-style=native
Note that if the repository gets out of whack, the following commands run on a linux box will correct things in the current working copy:
find | grep -v \._ | \
grep "\.\(js\|php\|css\|inc\|html\|html\|php5\|py\|json\|ini\|xml\|csv\)"\$ | \
xargs svn propset svn:eol-style native
find | grep -v \._ | \
grep "\.\(js\|php\|css\|inc\|html\|html\|php5\|py\|json\|ini\|xml\|csv\)"\$ | \
xargs dos2unix
### Feature Branches
For more complicated bugfixes or longer-term development, you may want to create a Feature Branch. For example, you might want
to add support for other rich-text-editors within the CMS - a complex task which can't be contained in a single patch.
Feature branches are a copy of trunk, and usually have a short lifetime in which active development happens.
**The feature branch maintainer is responsible for keeping his branch in sync with trunk and reintegrate when development
is complete.**
More information about ["Feature Branches"](http://svnbook.red-bean.com/en/1.5/svn.branchmerge.commonpatterns.html#svn.branchmerge.commonpatterns.feature),
[merging changes](http://svnbook.red-bean.com/en/1.5/svn.branchmerge.html) and [resolving conflicts](http://svnbook.red-bean.com/en/1.5/svn.tour.cycle.html#svn.tour.cycle.resolve).
#### Example: The 'nestedurls' branch
Example for a feature branch for the ''sapphire'' module called ''nestedurls''.
Creating the branch is a simple matter of running the ''svn cp'' command (see [SVN Book: "Creating a Branch"](http://svnbook.red-bean.com/en/1.5/svn.branchmerge.using.html#svn.branchmerge.using.create)).
svn cp http://svn.silverstripe.com/open/modules/sapphire/trunk http://svn.silverstripe.com/open/modules/sapphire/branches/nestedurls
After creating a feature branch, you commit your changes as usual, but also merge in any new changes from trunk
(see [SVN Book: "Keeping your Branch in Sync"](http://svnbook.red-bean.com/en/1.5/svn.branchmerge.basicmerging.html#svn.branchemerge.basicmerging.stayinsync)).
cd /your/nestedurls/working/copy
svn merge http://svn.silverstripe.com/open/modules/sapphire/trunk
Once you've finished your feature development (usually a timeframe around 4-8 weeks), you "reintegrate" your branch with the
trunk repository. This activity happens only once, and the feature branch has to be removed afterwards.
cd /your/trunk/working/copy
svn merge --reintegrate http://svn.silverstripe.com/open/modules/sapphire/branches/nestedurls
You can get information about the merge process (see
[SVN Book: "Mergeinfo and Previews"](http://svnbook.red-bean.com/en/1.5/svn.branchmerge.basicmerging.html#svn.branchmerge.basicmerging.mergeinfo)).
cd /your/nestedurls/working/copy
# revisions which are you still have to merge from trunk to your branch
svn mergeinfo http://svn.silverstripe.com/open/modules/sapphire/trunk --show-revs eligible
# revisions which are already merged from trunk to your branch
svn mergeinfo http://svn.silverstripe.com/open/modules/sapphire/trunk
### Troubleshooting
#### SVN for your own websites
Here is a step-by-step guide on how to work with SVN on your own site. It oversimplifies some aspects, but it is a good
introduction. NOTE: you will need SSH and SVN installed and available on your server.
* Install LAMP / WAMP and an SVN application (Tortoise SVN on Windows)
* Buy an SVN repository: http://www.SVNrepository.com/ OR set one up on your own server or look at freeby alternatives.
* Go to your SVN repository server and create a new repository - I would recommend to name it after the site you are about to setup, e.g. myfirstsite.com.
* Create / go to web folder on local LAMP/WAMP
* SVN "checkout" your empty repository (e.g. http://sunny.SVNrepository.com/SVN/myaccountname/myfirstsite.com).
* SVN "propedit" SVN:externals, add the following SVN property to the root directory:
SVN:externals (also add your own general modules here, etc... etc... this should be a long list):
cms http://SVN.silverstripe.com/open/modules/cms/branches/2.4
sapphire http://SVN.silverstripe.com/open/modules/sapphire/branches/2.4
* SVN "update" your project. All the various files should be imported now.
* SVN "commit" your externals.
* In the root directory, create the following files: _ss_environment.php.sample, .htacess.sample and "commit" these to your repository.
* Copy in local LAMP / WAMP environment: _ss_environment.php.sample to _ss_environment.php; and .htacess.sample to .htacess
* Make sure that .htaccess and _ss_environment.php files are excluded from any commits. This is another SVN property attached to the root directory:
SVN:ignore:
.htaccess
_ss_environment.php
assets
* Create assets folder in root directory
* Your site should now be ready on your local WAMP / LAMP. You can now create a mysite and themes folder, add all your files, edit, bla bla until your site is ready. Then SVN "add" and SVN "commit" all your files to your repository (usually, you do many commits)
* Once you have created the site locally and committed it to your repository you should SSH to your web server (recommended application for windows is PUTTY)
* Once logged in to your web server, browse to the root web folder
* Make sure SVN is installed on the server (just type SVN help or something to check).
* SSH prompt, type:
SVN checkout http://sunny.SVNrepository.com/SVN/myaccountname/myfirstsite.com .
<div class="hint" markdown="1">
Add a DOT at the end to check out files to current directory
</div>
* You should now have all your files on your webserver
* Copy on the server (using SSH)
cp _ss_environment.php.sample _ss_environment.php
cp .htacess.sample .htacess
* Edit these files: _ss_environment.php.sample , .htacess.sample, using the following SSH commands (if the nano application is not available then try pico):
nano _ss_environment.php.sample
nano .htacess.sample
* Create a folder called assets and make sure permissions are set correctly
* Website should now be up and running. (run dev/build to start).
#### A few point additional points:
* The whole concept of tags and branches have been left out, just to simplify.
* We have also left out the idea of a test and live site. The instructions here can be used to setup a test site. Once you are happy with a test site you can move the code to a live site using the SVN "merge" command. In principle, this is how it works: open SSH and go to the root directory of the live site. Then type:
svn merge http://mysvnrepository/branches/live/ http://mysvnrepository/branches/test/
* If you want to update a repository, but you want the repository on the webserver to be locked to a version then you need set the svn:externals as follows:
cms -r1234567 http://svn.silverstripe.com/open/modules/cms/branches/2.4
where 1234567 is the revision of your repository that you want to use
* If you want to get a better understanding of what is going on in a repository then use the following SVN commands: SVN "status" and SVN "info".
* You can not and should not make any changes to any of the core modules and other modules added with svn:externals
### Related
* [contributing](contributing)
* [release process](release-process)<file_sep>/docs/en/reference/director.md
# Director
## Introduction
`[api:Director]` is the first step in the "execution pipeline". It parses the URL, matching it to one of a number of patterns,
and determines the controller, action and any argument to be used. It then runs the controller, which will finally run
the viewer and/or perform processing steps.
## Best Practices
* Checking for an Ajax-Request: Use Director::is_ajax() instead of checking for $_REQUEST['ajax'].
## Redirection
The `[api:Director]` class has a number of methods to facilitate 301 and 302 HTTP redirection.
* **Director::redirect("action-name")**: If there's no slash in the URL passed to redirect, then it is assumed that you
want to go to a different action on the current controller.
* **Director::redirect("relative/url")**: If there is a slash in the URL, it's taken to be a normal URL. Relative URLs
will are assumed to be relative to the site-root; so Director::redirect("home/") will work no matter what the current
URL is.
* **Director::redirect("http://www.absoluteurl.com")**: Of course, you can pass redirect() absolute URL s too.
* **Director::redirectPerm("any-url")**: redirectPerm takes the same arguments as redirect, but it will send a 301
(permanent) instead of a 302 (temporary) header. It improves search rankings, so this should be used whenever the
following two conditions are true:
* Nothing happens server-side prior to the redirection
* The redirection will always occur
* **Director::redirectBack()**: This will return you to the previous page. There's no permanent version of
redirectBack().
## Custom Rewrite Rules
You can influence the way URLs are resolved one of 2 ways
1. Adding rules to `[api:Director]` in `<yourproject>/_config.php` (See Default Rewrite Rules below for examples)
2. Adding rules in your extended `[api:Controller]` class via the *$url_handlers* static variable
See [controller](/topics/controller) for examples and explanations on how the rules get processed for both 1 and 2 above.
* Static redirect for specific URL
:::php
Director::addRules(100, array(
'myPermanentRedirect' => 'redirect:http://www.mysite.com'
));
## Default Rewrite Rules
SilverStripe comes with certain rewrite rules (e.g. for *admin/assets*).
* [framework/_config.php](https://github.com/silverstripe/sapphire/blob/master/_config.php)
* [cms/_config.php](https://github.com/silverstripe/silverstripe-cms/blob/master/_config.php)
## Links
* See `[api:ModelAsController]` class for details on controller/model-coupling
* See [execution-pipeline](/reference/execution-pipeline) for custom routing
## API Documentation
`[api:Director]`
<file_sep>/docs/en/topics/grid-field.md
# Using and extending GridField
The `GridField` is a flexible form field for creating tables of data. It's new in SilverStripe 3.0 and replaces `ComplexTableField`, `TableListField`, and `TableField`. It's built as a lean core with a number of components that you plug into it. By selecting from the components that we provide or writing your own, you can grid a wide variety of grid controls.
## Using GridField
A GridField is created like any other field: you create an instance of the GridField object and add it to the fields of a form. At its simplest, GridField takes 3 arguments: field name, field title, and an `SS_List` of records to display.
This example might come from a Controller designed to manage the members of a group:
:::php
/**
* Form to display all members in a group
*/
public function MemberForm() {
$field = new GridField("Members", "Members of this group", $this->group->Members());
return new Form("MemberForm", $this, new FieldSet($field), new FieldSet());
}
Note that the only way to specify the data that is listed in a grid field is with `SS_List` argument. If you want to customise the data displayed, you can do so by customising this object.
This will create a read-only grid field that will show the columns specified in the Member's `$summary_fields` setting, and will let you sort and/or filter by those columns, as well as show pagination controls with a handful of records per page.
## GridFieldConfig: Portable configuration
The example above a useful default case, but when developing applications you may need to control the behaviour of your grid more precisely than this. To this end, the `GridField` constructor allows for fourth argument, `$config`, where you can pass a `GridFieldConfig` object.
This example creates exactly the same kind of grid as the previous example, but it creates the configuration manually:
:::php
$config = GridFieldConfig::create();
// Provide a header row with filter controls
$config->addComponent(new GridFieldFilterHeader());
// Provide a default set of columns based on $summary_fields
$config->addComponent(new GridFieldDataColumns());
// Provide a header row with sort controls
$config->addComponent(new GridFieldSortableHeader());
// Paginate results to 25 items per page, and show a footer with pagination controls
$config->addComponent(new GridFieldPaginator(25));
$field = new GridField("Members", "Members of this group", $this->group->Members(), $config);
If we wanted to make a simpler grid without pagination or filtering, we could do so like this:
:::php
$config = GridFieldConfig::create();
// Provide a default set of columns based on $summary_fields
$config->addComponent(new GridFieldDataColumns());
// Provide a header row with sort controls
$config->addComponent(new GridFieldPaginator(25));
$field = new GridField("Members", "Members of this group", $this->group->Members(), $config);
A `GridFieldConfig` is made up of a new of `GridFieldComponent` objects, which are described in the next chapter.
## GridFieldComponent: Modular features
`GridFieldComponent` is a family of interfaces.
SilverStripe Framework comes with the following components that you can use out of the box.
### GridFieldDataColumns
This is the one component that, in most cases, you must include. It provides the default columns, sourcing them from the underlying DataObject's `$summary_fields` if no specific configuration is provided.
Without GridFieldDataColumns added to a GridField, it would have no columns whatsoever. Although this isn't particularly useful most of the time, we have allowed for this for two reasons:
* You may have a grid whose fields are generated purely by another non-standard component.
* It keeps the core of the GridField lean, focused solely on providing APIs to the components.
There are a number of methods that can be called on GridField to configure its behaviour.
You can choose which fields you wish to display:
:::php
$gridField->setDisplayFields(array(
'ID' => 'ID',
'FirstName' => 'First name',
'Surname' => 'Surname',
'Email' => 'Email',
'LastVisited' => 'Last visited',
));
You can specify formatting operations, for example choosing the format in which a date is displayed:
:::php
$gridField->setFieldCasting(array(
'LastVisited' => 'Date->Ago',
));
You can also specify formatting replacements, to replace column contents with HTML tags:
:::php
$gridField->setFieldFormatting(array(
'Email' => '<strong>$Email</strong>',
));
**EXPERIMENTAL API WARNING:** We will most likely refactor this so that this configuration methods are called on the component rather than the grid field.
### GridFieldSortableHeader
This component will add a header to the grid with sort buttons. It will detect which columns are sortable and only provide sort controls on those columns.
### GridFieldFilterHeader
This component will add a header row with a text field filter for each column, letting you filter the results with text searches. It will detect which columns are filterable and only provide filter controls on those columns.
### GridFieldPaginator
This component will limit output to a fixed number of items per page add a footer row with pagination controls. The constructor takes 1 argument: the number of items per page.
### GridFieldDeleteButton
TODO Describe component
### GridFieldEditButton
Adds a edit button to each row of the table. This needs another component to provide an edit interface - see GridFieldDetailForm for use within the CMS.
### GridFieldRelationAdd
This class is is responsible for adding objects to another object's has_many and many_many relation,
as defined by the `[api:RelationList]` passed to the GridField constructor.
Objects can be searched through an input field (partially matching one or more fields).
Selecting from the results will add the object to the relation.
Often used alongside `[api:GridFieldRemoveButton]` for detaching existing records from a relatinship.
For easier setup, have a look at a sample configuration in `[api:GridFieldConfig_RelationEditor]`.
### GridFieldRemoveButton
Allows to detach an item from an existing has_many or many_many relationship.
Similar to {@link GridFieldDeleteAction}, but allows to distinguish between
a "delete" and "detach" action in the UI - and to use both in parallel, if required.
Requires the GridField to be populated with a `[api:RelationList]` rather than a plain DataList.
Often used alongside `[api:GridFieldAddExistingAutocompleter]` to add existing records to the relationship.
### GridFieldDetailForm
Provides add and edit forms for use within the CMS. This allows editing of the linked records.
This only provides the actual add/edit forms, GridFieldEditButton is required to provide a button to link to the edit form,
and GridFieldToolbarHeader is required to provide an add button.
### GridFieldToolbarHeader
Adds a title bar to the top of the GridField, with optional "New" button. The New button doesn't provide any functionality with this component alone - see GridFieldDetailForm.
### GridFieldExportButton
Adds an "Download as CSV" button. This will save the current List shown in the GridField as CSV. Takes the
## Extending GridField with custom components
You can create a custom component by building a class that implements one or more of the following interfaces: `GridField_HTMLProvider`, `GridField_ColumnProvider`, `GridField_ActionProvider`, or `GridField_DataManipulator`.
All of the methods expected by these interfaces take `$gridField` as their first argument. The gridField related to the component isn't set as a property of the component instance. This means that you can re-use the same component object across multiple `GridField`s, if that is appropriate.
It's common for a component to implement several of these interfaces in order to provide the complete implementation of a feature. For example, `GridFieldSortableHeader` implements the following:
* `GridField_HTMLProvider`, to generate the header row including the GridField_Action buttons
* `GridField_ActionProvider`, to define the sortasc and sortdesc actions that add sort column and direction to the state.
* `GridField_DataManipulator`, to alter the sorting of the data list based on the sort column and direction values in the state.
### GridFieldAddExistingAutocompleter
A GridFieldAddExistingAutocompleter is responsible for adding objects to another object's `has_many` and `many_many` relation,
as defined by the `[api:RelationList]` passed to the GridField constructor.
Objects can be searched through an input field (partially matching one or more fields).
Selecting from the results will add the object to the relation.
:::php
$group = DataObject::get_one('Group');
$config = GridFieldConfig::create()->addComponent(new GridFieldAddExistingAutocompleter(array('FirstName', 'Surname', 'Email'));
$gridField = new GridField('Members', 'Members', $group->Members(), $config);
## Component interfaces
### GridField_HTMLProvider
The core GridField provides the following basic HTML:
* A `<table>`, with an empty `<thead>` and `<tfoot>`
* A collection of `<tr>`s, based on the grid's data list, each of which will contain a collection or `<td>`s based on the grid's columns.
The `GridField_HTMLProvider` component can provide HTML that goes into the `<thead>` or `<tfoot>`, or that appears before or after the table itself.
It should define the getHTMLFragments() method, which should return a map. The map keys are can be 'header', 'footer', 'before', or 'after'. The map values should be strings containing the HTML content to put into each of these spots. Only the keys for which you wish to provide content need to be defined.
For example, this components will add a footer row to the grid field, thanking the user for their patronage. You can see that we make use of `$gridField->getColumnCount()` to ensure that the single-cell row takes up the full width of the grid.
:::php
class ThankYouForUsingSilverStripe implements GridField_HTMLProvider {
public function getHTMLFragments($gridField) {
$colSpan = $gridField->getColumnCount();
return array(
'footer' => '<tr><td colspan="' . $colSpan . '">Thank you for using SilverStripe!</td></tr>',
);
}
}
If you wish to add CSS or JavaScript for your component, you may also make `Requirements` calls in this method.
### Defining new fragments
Sometimes it is helpful to have one component write HTML into another component. For example, you might have an action header row at the top of your GridField that several different components may define actions for.
To do this, you can put the following code into one of the HTML fragments returned by an HTML provider.
$DefineFragment(fragment-name)
Other `GridField_HTMLProvider` components can now write to `fragment-name` just as they would write to footer, etc. Fragments can be nested.
For example, this component creates a `header-actions` fragment name that can be populated by other components:
:::php
class HeaderActionComponent implements GridField_HTMLProvider {
public function getHTMLFragments($gridField) {
$colSpan = $gridField->getColumnCount();
array(
"header" => "<tr><td colspan=\"$colspan\">\$DefineFragment(header-actions)</td></tr>"
);
}
}
This is a simple example of how you might populate that new fragment:
:::php
class AddNewActionComponent implements GridField_HTMLProvider {
public function getHTMLFragments($gridField) {
$colSpan = $gridField->getColumnCount();
array(
"header-actions" => "<button>Add new</button>"
);
}
}
If you write to a fragment that isn't defined anywhere, or you create a circular dependency within fragments, an exception will be thrown.
### GridField_ColumnProvider
By default, a grid contains no columns. All the columns displayed in a grid will need to be added by an appropriate component.
For example, you may create a grid field with several components providing columns:
* `GridFieldDataColumns` could provide basic data columns.
* An editor component could provide a column containing action buttons on the right.
* A multiselect component clould provide a column showing a checkbox on the left.
In order to provide additional columns, your component must implement `GridField_ColumnProvider`.
First you need to define 2 methods that specify which columns need to be added:
* **`function augmentColumns($gridField, &$columns)`:** Update the `$columns` variable (passed by reference) to include the names of the additional columns that this component provides. You can insert the values at any point you wish, for example if you need to add a column to the left of the grid, rather than the right.
* **`function getColumnsHandled($gridField)`:** Return an array of the column names. This overlaps with the function of `augmentColumns()` but leaves out any information about the order in which the columns are added.
Then you define 3 methods that specify what should be shown in these columns:
* **`function getColumnContent($gridField, $record, $columnName)`:** Return the HTML content of this column for the given record. Like `GridField_HTMLProvider`, you may make `Requirements` calls in this method.
* **`function getColumnAttributes($gridField, $record, $columnName)`:** Return a map of the HTML attributes to add to this column's `<td>` for this record. Most commonly, this is used to specify a colspan.
* **`function getColumnMetadata($gridField, $columnName)`:** Return a map of the metadata about this column. Right now, only one piece of meta-data is specified, "title". Other components (such as those responsible for generating headers) may fetch the column meta-data for their own purposes.
### GridField_ActionProvider
Most grid fields worthy of the name are interactive in some way. Users might able to page between results, sort by different columns, filter the results or delete records. Where this interaction necessitates an action on the server side, the following generally happens:
* The user triggers an action.
* That action updates the state, database, or something else.
* The GridField is re-rendered with that new state.
These actions can be provided by components that implement the `GridField_ActionProvider` interface.
An action is defined by two things: an action name, and zero or more named arguments. There is no built-in notion of a record-specific or column-specific action, but you may choose to define an argument such as ColumnName or RecordID in order to implement these.
To provide your actions, define the following two functions:
* **`function getActions($gridField)`:** Return a list of actions that this component provides. There is no namespacing on these actions, so you need to ensure that they don't conflict with other components.
* **`function handleAction(GridField $gridField, $actionName, $arguments, $data)`:** Handle the action defined by `$actionName` and `$arguments`. `$data` will contain the full data from the form, if you need to access that.
To call your actions, you need to create `GridField_FormAction` elsewhere in your component. Read more about them below.
**EXPERIMENTAL API WARNING:** handleAction implementations often contain a big switch statement and this interface might be amended on, such that each action is defined in a separate method. If we do this, it will be done before 3.0 stable so that we can lock down the API, but early adopters should be aware of this potential for change!
### GridField_DataManipulator
A `GridField_DataManipulator` component can modify the data list. For example, a paginating component can apply a limit, or a sorting component can apply a sort. Generally, the data manipulator will make use of to `GridState` variables to decide how to modify the data list (see GridState below).
* **`getManipulatedData(GridField $gridField, SS_List $dataList)`:** Given this grid's data list, return an updated list to be used with this grid.
### GridField_URLHandler
Sometimes an action isn't enough: you need to provide additional support URLs for the grid. These URLs may return user-visible content, for example a pop-up form for editing a record's details, or they may be support URLs for front-end functionality, for example a URL that will return JSON-formatted data for a javascript grid control.
To build these components, you should implement the `GridField_URLHandler` interface. It only specifies one method: `getURLHandlers($gridField)`. This method should return an array similar to the `RequestHandler::$url_handlers` static. The action handlers should also be defined on the component; they will be passed `$gridField` and `$request`.
Here is an example in full. The actual implementation of the view and edit forms isn't included.
:::php
/**
* Provides view and edit forms at GridField-specific URLs. These can be placed into pop-ups by an appropriate front-end.
*
* The URLs provided will be off the following form:
* - <FormURL>/field/<GridFieldName>/item/<RecordID>
* - <FormURL>/field/<GridFieldName>/item/<RecordID>/edit
*/
class GridFieldDetailForm implements GridField_URLHandler {
public function getURLHandlers($gridField) {
return array(
'item/$ID' => 'handleItem',
);
}
public function handleItem($gridField, $request) {
$record = $gridField->getList()->byId($request->param("ID"));
return new GridFieldDetailForm_ItemRequest($gridField, $this, $record);
}
}
class GridFieldDetailForm_ItemRequest extends RequestHandler {
protected $gridField;
protected $component;
protected $record;
public function __construct($gridField, $component, $record) {
$this->gridField = $gridField;
$this->component = $gridField;
$this->record = $record;
parent::__construct();
}
public function index() {
echo "view form for record #" . $record->ID;
}
public function edit() {
echo "edit form for record #" . $record->ID;
}
}
## Other tools
### GridState
Each `GridField` object has a key-store available handled by the `GridState` class. You can call `$gridField->State` to get access to this key-store. You may reference any key name you like, and do so recursively to any depth you like:
:::php
$gridField->State->Foo->Bar->Something = "hello";
Because there is no schema for the grid state, its good practice to keep your state within a namespace, by first accessing a state key that has the same name as your component class. For example, this is how the `GridFieldSortableHeader` component manages its sort state.
:::php
$state = $gridField->State->GridFieldSortableHeader;
$state->SortColumn = $arguments['SortColumn'];
$state->SortDirection = 'asc';
...
$state = $gridField->State->GridFieldSortableHeader;
if ($state->SortColumn == "") {
return $dataList;
} else {
return $dataList->sort($state->SortColumn, $state->SortDirection)
}
When checking for empty values in the state, you should compare the state value to the empty string. This is because state values always return a `GridState_Data` object, and comparing to an empty string will call its `__toString()` method.
:::php
// Good
if ($state->SortColumn == "") { ... }
// Bad
if (!$state->SortColumn) { ... }
**NOTE:** Under the hood, `GridState` is a subclass of hidden field that provides a `getData()` method that returns a `GridState_Data` object. `$gridField->getState()` returns that `GridState_Data` object.
### GridField_Action
The `GridField_Action` class is a subclass of `FormAction` that will provide a button designed to trigger a grid field action. This is how you can link user-interface controls to the actions defined in `GridField_ActionProvider` components.
To create the action button, instantiate the object with the following arguments to your constructor:
* grid field
* button name
* button label
* action name
* action arguments (an array of named arguments)
For example, this could be used to create a sort button:
:::php
$field = new GridField_Action(
$gridField, 'SetOrder'.$columnField, $title,
"sortasc", array('SortColumn' => $columnField));
Once you have created your button, you need to render it somewhere. You can include the `GridField_Action` object in a template that is being rendered, or you can call its `Field()` method to generate the HTML content.
:::php
$output .= $field->Field();
Most likely, you will do this in `GridField_HTMLProvider::getHTMLFragments()` or `GridField_ColumnProvider::getColumnContent()`.
### GridField Helper Methods
The GridField class provides a number of methods that are useful for components. See [the API documentation](api:GridField) for the full list, but here are a few:
* **`getList()`:** Returns the data list for this grid, without the state modifications applied.
* **`getState()`:** Also called as `$gridField->State`, returns the `GridState_Data` object storing the current state.
* **`getColumnMetadata($column)`:** Return the metadata of the given column.
* **`getColumnCount()`:** Returns the number of columns<file_sep>/docs/en/reference/dataobjectset.md
# DataObjectSet
## Introduction
This class represents a set of `[api:DataObject]`s, such as the results of a query. It is the base for all
[datamodel](/topics/datamodel)-related querying. It implements the [Iterator
interface](http://php.net/manual/en/language.oop5.iterations.php) introduced in PHP5.
Relations (`has_many`/`many_many`) are described in `[api:ComponentSet]`, a subclass of `[api:DataObjectSet]`.
## Usage
### Getting the size
:::php
$mySet->Count();
### Getting an single element
:::php
$myFirstDataObject = $mySet->First();
$myLastDataObject = $mySet->Last();
### Getting multiple elements
:::php
$mySpecialDataObjects = $mySet->find('Status', 'special');
$startingFromTen = $mySet->getOffset(10);
$tenToTwenty = $mySet->getRange(10, 10);
### Getting one property
:::php
$myIDArray = $mySet->column('ID');
### Grouping
You can group a set by a specific column. Consider using `[api:SQLQuery]` with a *GROUP BY* statement for enhanced
performance.
:::php
$groupedSet = $mySet->groupBy('Lastname');
### Sorting
Sort a set by a specific column.
:::php
$mySet->sort('Lastname'); //ascending
$mySet->sort('Lastname', 'DESC'); //descending
This works on the object itself, so do NOT do something like this:
:::php
$sortedSet = $mySet->sort('Lastname'); //ascending
## Merge with other `[api:DataObjectSet]`s
:::php
$myFirstSet->merge($mySecondSet);
// $myFirstSet now contains all combined values
### Mapping for Dropdowns
When using `[api:DropdownField]` and its numerous subclasses to select a value from a set, you can easily map
the records to a compatible array:
:::php
$map = $mySet->toDropDownMap('ID', 'Title');
$dropdownField = new DropdownField('myField', 'my label', $map);
### Converting to array
:::php
$myArray = $mySet->toArray();
### Checking for existence
It is good practice to check for empty sets before doing any iteration.
:::php
$mySet = DataObject::get('Players');
if($mySet->exists()) foreach($mySet as $player)
### Paging
`[api:DataObject]`s have native support for dealing with **pagination**.
See *setPageLimits*, *setPageLength*, etc.
FIXME Complete pagination documentation
## API Documentation
`[api:DataObjectSet]`
<file_sep>/docs/en/tutorials/4-site-search.md
# Tutorial 4 - Site Search
## Overview
This is a short tutorial demonstrating how to add search functionality to a SilverStripe site. It is recommended that
you have completed the earlier tutorials, especially the tutorial on forms, before attempting this tutorial. While this
tutorial will add search functionality to the site built in the previous tutorials, it should be straight forward to
follow this tutorial on any site of your own.
## What are we working towards?
We are going to add a search box on the top of the page. When a user types something in the box, they are taken to a
results page.

## Creating the search form
To enable the search engine you need to include the following code in your `mysite/_config.php` file.
This will enable fulltext search on page content as well as names of all files in the `/assets` folder.
:::php
FulltextSearchable::enable();
After including that in your `_config.php` you will need to rebuild the database by visiting `http://yoursite.com/dev/build` in your web browser. This will add the fulltext search columns.
The actual search form code is already provided in FulltextSearchable so when you add the enable line above to your
`_config.php` you can add your form as `$SearchForm`.
### Custom CSS code
Add the following css code to the *themes/tutorial/css/layout.css* file. This will style the header form and search
results page.
:::css
#Header form {
float:right;
width:160px;
margin:25px 25px 0px 25px;
}
#Header form * {
display:inline !important;
}
#Header form div {
}
#Header form input.text {
width:110px;
color:#000;
background:#f0f0f0;
border:1px solid #aaa;
padding:3px;
}
#Header form input.action {
font-weight:bold;
}
.searchResults h2 {
font-size:2.2em;
font-weight:normal;
color:#0083C8;
margin-bottom:15px;
}
.searchResults p.searchQuery {
color:#333;
margin-bottom:10px;
}
.searchResults ul#SearchResults li {
margin-bottom:20px;
}
ul#SearchResults p {
font-size:1.1em;
font-weight:normal;
line-height:2em;
color:#333;
}
ul#SearchResults a.searchResultHeader {
font-size:1.3em;
font-weight:bold;
color:#0083C8;
text-decoration:none;
margin:20px 0 8px 0;
padding-left:20px;
background:url(../images/treeicons/search-file.gif) no-repeat left center;
}
ul#SearchResults a {
text-decoration:none;
color:#0083C8;
}
ul#SearchResults a:hover {
border-bottom:1px dotted #0083C8;
}
## Adding the search form
We then just need to add the search form to the template. Add *$SearchForm* to the 'Header' div in
*themes/tutorial/templates/Page.ss*.
*themes/tutorial/templates/Page.ss*
:::ss
<div id="Header">
$SearchForm
<h1>$Title</h1>
</div>

## Showing the results
Next we need to create the *results* function.
*mysite/code/Page.php*
:::php
class Page_Controller extends ContentController {
...
public function results($data, $form){
$data = array(
'Results' => $form->getResults(),
'Query' => $form->getSearchQuery(),
'Title' => 'Search Results'
);
$this->Query = $form->getSearchQuery();
return $this->customise($data)->renderWith(array('Page_results', 'Page'));
}
}
First we populate an array with the data we wish to pass to the template - the search results, query and title of the
page. The final line is a little more complicated.
When we call a function by its url (eg http://localhost/home/results), SilverStripe will look for a template with the
name `PageType_function.ss`. As we are implementing the *results* function on the *Page* page type, we create our
results page template as *Page_results.ss*. Unfortunately this doesn't work when we are using page types that are
children of the *Page* page type. For example, if someone used the search on the homepage, it would be rendered with
*Homepage.ss* rather than *Page_results.ss*. SilverStripe always looks for the template from the most specific page type
first, so in this case it would use the first template it finds in this list:
* HomePage_results.ss
* HomePage.ss
* Page_results.ss
* Page.ss
We can override this list by using the *renderWith* function. The *renderWith* function takes an array of the names of
the templates you wish to render the page with. Here we first add the data to the page by using the 'customise'
function, and then attempt to render it with *Page_results.ss*, falling back to *Page.ss* if there is no
*Page_results.ss*.
## Creating the template
Lastly we need to create the template for the search page. This template uses all the same techniques used in previous
tutorials. It also uses a number of pagination variables, which are provided by the `[api:DataObjectSet]`
class.
*themes/tutorial/templates/Layout/Page_results.ss*
:::ss
<div id="Content" class="searchResults">
<h2>$Title</h2>
<% if Query %>
<p class="searchQuery"><strong>You searched for "{$Query}"</strong></p>
<% end_if %>
<% if Results %>
<ul id="SearchResults">
<% control Results %>
<li>
<a class="searchResultHeader" href="$Link">
<% if MenuTitle %>
$MenuTitle
<% else %>
$Title
<% end_if %>
</a>
<p>$Content.LimitWordCountXML</p>
<a class="readMoreLink" href="$Link" title="Read more about "{$Title}"">Read more about "{$Title}"...</a>
</li>
<% end_control %>
</ul>
<% else %>
<p>Sorry, your search query did not return any results.</p>
<% end_if %>
<% if Results.MoreThanOnePage %>
<div id="PageNumbers">
<% if Results.NotLastPage %>
<a class="next" href="$Results.NextLink" title="View the next page">Next</a>
<% end_if %>
<% if Results.NotFirstPage %>
<a class="prev" href="$Results.PrevLink" title="View the previous page">Prev</a>
<% end_if %>
<span>
<% control Results.Pages %>
<% if CurrentBool %>
$PageNum
<% else %>
<a href="$Link" title="View page number $PageNum">$PageNum</a>
<% end_if %>
<% end_control %>
</span>
<p>Page $Results.CurrentPage of $Results.TotalPages</p>
</div>
<% end_if %>
</div>
Then finally add ?flush=1 to the URL and you should see the new template.

## Summary
This tutorial has demonstrated how easy it is to have full text searching on your site. To add search to a SilverStripe
site, only a search form and a results page need to be created.
[Next Tutorial >>](5-dataobject-relationship-management)
<file_sep>/tests/control/PjaxResponseNegotiatorTest.php
<?php
class PjaxResponseNegotiatorTest extends SapphireTest {
function testDefaultCallbacks() {
$negotiator = new PjaxResponseNegotiator(array(
'default' => function() {return 'default response';},
));
$request = new SS_HTTPRequest('GET', '/'); // not setting pjax header
$this->assertEquals('default response', $negotiator->respond($request));
}
function testSelectsFragmentByHeader() {
$negotiator = new PjaxResponseNegotiator(array(
'default' => function() {return 'default response';},
'myfragment' => function() {return 'myfragment response';},
));
$request = new SS_HTTPRequest('GET', '/');
$request->addHeader('X-Pjax', 'myfragment');
$this->assertEquals('myfragment response', $negotiator->respond($request));
}
}
<file_sep>/docs/en/installation/upgrading.md
# Upgrading
Usually an update or upgrade your SilverStripe installation just means overwriting files and updating your
database-schema. Please see your [upgrade notes and changelogs](/changelogs).
## Process
Never update a website on the live server without trying it on a development copy first.
* Check if any modules (e.g. blog or forum) in your installation are compatible and need to be upgraded as well
* Backup your database
* Backup your website
* Download the new release and uncompress it to a temporary folder
* Leave custom folders like *mysite* or *themes* in place.
* Identify system folders in your webroot (`cms`, `framework` and any additional modules).
* Delete existing system folders (or move them outside of your webroot)
* Extract and replace system folders from your download (Deleting instead of "copying over" existing folders
ensures that files removed from the new SilverStripe release are not persisting in your installation)
* Visit http://yoursite.com/dev/build/?flush=1 to rebuild the website Database
* Check if you need to adapt your code to changed APIs
* Check if you need to adapt your code to changed CSS/HTML/JS
* See [common-problems](common-problems) for a list of likely mistakes that could happen during an upgrade.
## Decision Helpers
How easy will it be to update my project? It's a fair question, and sometimes a difficult one to answer. This page is
intended to help you work out how hard it will be to upgrade your site.
* If you've made custom branches of the core, or of a module, it's going to be harder to upgrade.
* The more custom features you have, the harder it will be to upgrade. You will have to re-test all of those features
and some of them may have broken.
* Customisations of a well defined type - such as custom page types or custom blog widgets - are going to be easier to
upgrade than customisations that use sneaky tricks, such as the subsites module.
## Related
* [Release Announcements](http://groups.google.com/group/silverstripe-announce/)
* [Blog posts about releases on silverstripe.org](http://silverstripe.org/blog/tag/release)
<file_sep>/docs/en/tutorials/3-forms.md
# Tutorial 3 - Forms
## Overview
This tutorial is intended to be a continuation of the first two tutorials, and will build on the site produced in those
two tutorials.
This tutorial explores forms in SilverStripe. It will look at coded forms. Forms which need to be written in PHP.
Another method which allows you to construct forms via the CMS is by using the [userforms module](http://silverstripe.org/user-forms-module).
A UserDefinedForm is much quicker to implement, but lacks the flexibility of a coded form.
## What are we working towards?
We will create a poll on the home page that asks the user their favourite web browser, and displays a bar graph of the
results.

## Creating the form
We will be creating a form for a poll on the home page.
The poll will ask the user's name and favourite web browser, and then collate the results into a bar graph. We create
the form in a method on *HomePage_Controller*.
*mysite/code/HomePage.php*
:::php
class HomePage_Controller extends Page_Controller {
// ...
public function BrowserPollForm() {
// Create fields
$fields = new FieldList(
new TextField('Name'),
new OptionsetField('Browser', 'Your Favourite Browser', array(
'Firefox' => 'Firefox',
'Chrome' => 'Chrome',
'Internet Explorer' => 'Internet Explorer',
'Safari' => 'Safari',
'Opera' => 'Opera',
'Lynx' => 'Lynx'
))
);
// Create actions
$actions = new FieldList(
new FormAction('doBrowserPoll', 'Submit')
);
return new Form($this, 'BrowserPollForm', $fields, $actions);
}
...
}
...
Let's step through this code.
:::php
// Create fields
$fields = new FieldList(
new TextField('Name'),
new OptionsetField('Browser', 'Your Favourite Browser', array(
'Firefox' => 'Firefox',
'Chrome' => 'Chrome',
'Internet Explorer' => 'Internet Explorer',
'Safari' => 'Safari',
'Opera' => 'Opera',
'Lynx' => 'Lynx'
))
);
First we create our form fields.
We do this by creating a `[api:FieldList]` and passing our fields as arguments. The first field is a new
`[api:TextField]` with the name 'Name'.
There is a second argument when creating a field which specifies the text on the label of the field. If no second
argument is passed, as in this case, it is assumed the label is the same as the name of the field.
The second field we create is an `[api:OptionsetField]`. This is a dropdown, and takes a third argument - an
array mapping the values to the options listed in the dropdown.
:::php
$actions = new FieldList(
new FormAction('doBrowserPoll', 'Submit');
);
After creating the fields, we create the form actions. Form actions appear as buttons at the bottom of the form.
The first argument is the name of the function to call when the button is pressed, and the second is the label of the
button.
Here we create a 'Submit' button which calls the 'doBrowserPoll' method, which we will create later.
All the form actions (in this case only one) are collected into a `[api:FieldList]` object the same way we did with
the fields.
:::php
return new Form($this, 'BrowserPollForm', $fields, $actions);
Finally we create the `[api:Form]` object and return it.
The first argument is the controller that contains the form, in most cases '$this'. The second is the name of the method
that returns the form, which is 'BrowserPollForm' in our case. The third and fourth arguments are the
FieldLists containing the fields and form actions respectively.
After creating the form function, we need to add the form to our home page template.
Add the following code to the home page template, just before the Content `<div>`:
*themes/tutorial/templates/Layout/HomePage.ss*
:::ss
...
<div id="BrowserPoll">
<h2>Browser Poll</h2>
$BrowserPollForm
</div>
<div id="Content">
...
Add the following code to the form style sheet:
*themes/tutorial/css/form.css*
:::css
/* BROWSER POLL */
#BrowserPoll {
float: right;
margin: 20px 10px 0 0;
width: 20%;
}
form FieldList {
border:0;
}
#BrowserPoll .message {
display: block;
color:red;
background:#ddd;
border:1px solid #ccc;
padding:5px;
margin:5px;
}
#BrowserPoll h2 {
font-size: 1.5em;
color: #0083C8;
}
#BrowserPoll .field {
padding:3px 0;
}
#BrowserPoll .Actions {
padding:5px 0;
}
#BrowserPoll .bar {
background-color: #015581;
}
This CSS code will ensure that the form is formatted and positioned correctly. All going according to plan, if you visit
[http://localhost/home?flush=1](http://localhost/home?flush=1) it should look something like below.

## Processing the form
Great! We now have a browser poll form, but it doesn't actually do anything. In order to make the form work, we have to
implement the 'doBrowserPoll' method that we told it about.
First, we need some way of saving the poll submissions to the database, so we can retrieve the results later. We can do
this by creating a new object that extends from `[api:DataObject]`.
If you recall, in tutorial two we said that all objects that inherit from DataObject and that add fields are stored in
the database. Also recall that all pages extend DataObject indirectly through `[api:SiteTree]`. Here instead of
extending SiteTree (or `[api:Page]`) to create a page type, we extend DataObject directly.
*mysite/code/BrowserPollSubmission.php*
:::php
<?php
class BrowserPollSubmission extends DataObject {
static $db = array(
'Name' => 'Text',
'Browser' => 'Text'
);
}
If we then rebuild the database ([http://localhost/db/build?flush=1](http://localhost/db/build?flush=1)), we will see
that the *BrowserPollSubmission* table is created. Now we just need to define 'doBrowserPoll' on *HomePage_Controller*.
*mysite/code/HomePage.php*
:::php
class HomePage_Controller extends Page_Controller {
// ...
public function doBrowserPoll($data, $form) {
$submission = new BrowserPollSubmission();
$form->saveInto($submission);
$submission->write();
Director::redirectBack();
}
}
A function that processes a form submission takes two arguments - the first is the data in the form, the second is the
`[api:Form]` object.
In our function we create a new *BrowserPollSubmission* object. Since the name of our form fields and the name of the
database fields are the same we can save the form directly into the data object.
We call the 'write' method to write our data to the database, and 'Director::redirectBack()' will redirect the user back
to the home page.
## Form validation
SilverStripe forms all have automatic validation on fields where it is logical. For example, all email fields check that
they contain a valid email address. You can write your own validation by subclassing the *Validator* class.
SilverStripe provides the *RequiredFields* validator, which ensures that the fields specified are filled in before the
form is submitted. To use it we create a new *RequiredFields* object with the name of the fields we wish to be required
as the arguments, then pass this as a fifth argument to the Form constructor.
Change the end of the 'BrowserPollForm' function so it looks like this:
** mysite/code/HomePage.php **
:::php
public function BrowserPollForm() {
...
// Create validator
$validator = new RequiredFields('Name', 'Browser');
return new Form($this, 'BrowserPollForm', $fields, $actions, $validator);
}
If we then open the homepage and attempt to submit the form without filling in the required fields an error will be
shown.

## Showing the poll results
Now that we have a working form, we need some way of showing the results.
The first thing to do is make it so a user can only vote once per session. If the user hasn't voted, show the form,
otherwise show the results.
We can do this using a session variable. The `[api:Session]` class handles all session variables in SilverStripe.
First modify the 'doBrowserPoll' to set the session variable 'BrowserPollVoted' when a user votes.
*mysite/code/HomePage.php*
:::php
...
HomePage_Controller extends Page_Controller {
...
public function doBrowserPoll($data, $form) {
$submission = new BrowserPollSubmission();
$form->saveInto($submission);
$submission->write();
Session::set('BrowserPollVoted', true);
Director::redirectBack();
}
...
}
Then we simply need to check if the session variable has been set in 'BrowserPollForm()', and to not return the form if
it is.
:::php
public function BrowserPollForm() {
if(Session::get('BrowserPollVoted')) {
return false;
}
...
If you visit the home page now you will see you can only vote once per session; after that the form won't be shown. You
can start a new session by closing and reopening your browser (or if you're using Firefox and have installed the [Web
Developer](http://chrispederick.com/work/web-developer/) extension, you can use its Clear Session Cookies command).
Although the form is not shown, you'll still see the 'Browser Poll' heading. We'll leave this for now: after we've built
the bar graph of the results, we'll modify the template to show the graph instead of the form if the user has already
voted.
We now need some way of getting the data from the database into the template.
In the second tutorial we got the latest news articles for the home page by using the 'DataObject::get' function. We
can't use the 'DataObject::get' function here directly as we wish to count the total number of votes for each browser.
By looking at the documentation for 'DataObject::get', we can see that it returns a `[api:DataObjectSet]`
object. In fact, all data that can be iterated over in a template with a page control is contained in a DataObjectSet.
A `[api:DataObjectSet]` is a set of not just DataObjects, but of ViewableData, which the majority of
SilverStripe's classes (including DataObject) inherit from. We can create a DataObjectSet, fill it with our data, and
then create our graph using a page control in the template. Create the function 'BrowserPollResults' on the
*HomePage_Controller* class.
** mysite/code/HomePage.php **
:::php
public function BrowserPollResults() {
$submissions = DataObject::get('BrowserPollSubmission');
$total = $submissions->Count();
$doSet = new DataObjectSet();
foreach($submissions->groupBy('Browser') as $browser => $data) {
$percentage = (int) ($data->Count() / $total * 100);
$record = array(
'Browser' => $browser,
'Percentage' => $percentage
);
$doSet->push(new ArrayData($record));
}
return $doSet;
}
This introduces a few new concepts, so let's step through it.
:::php
$submissions = DataObject::get('BrowserPollSubmission');
First we get all of the *BrowserPollSubmission*s from the database. This returns the submissions as a
DataObjectSet, which contains the submissions as *BrowserPollSubmission* objects.
:::php
$total = $submissions->Count();
We get the total number of submissions, which is needed to calculate the percentages.
:::php
$doSet = new DataObjectSet();
foreach($submissions->groupBy('Browser') as $browser => $data) {
$percentage = (int) ($data->Count() / $total * 100);
$record = array(
'Browser' => $browser,
'Percentage' => $percentage
);
$doSet->push(new ArrayData($record));
}
Now we create an empty DataObjectSet to hold our data and then iterate over the 'Browser' submissions field. The 'groupBy'
method of DataObjectSet splits our DataObjectSet by the 'Browser' field passed to it. The percentage of submissions for each
browser is calculated using the size of the DataObjectSet. It puts these new DataObjectSets into an array indexed
by the value of the field. The `[api:ArrayData]` class wraps an array into a ViewableData object, so we finally create a new
ArrayData object, which we can add to our *$doSet* DataObjectSet of results.
:::php
return $doSet;
After we have iterated through all the browsers, the DataObjectSet contains all the results, which is then
returned.
The final step is to create the template to display our data. Change the 'BrowserPoll' div in
*themes/tutorial/templates/Layout/HomePage.ss* to the below.
:::ss
<div id="BrowserPoll">
<h2>Browser Poll</h2>
<% if BrowserPollForm %>
$BrowserPollForm
<% else %>
<ul>
<% control BrowserPollResults %>
<li>
<div class="browser">$Browser: $Percentage%</div>
<div class="bar" style="width:$Percentage%"> </div>
</li>
<% end_control %>
</ul>
<% end_if %>
</div>
Here we first check if the *BrowserPollForm* is returned, and if it is display it. Otherwise the user has already voted,
and the poll results need to be displayed.
We use the normal tactic of putting the data into an unordered list and using CSS to style it, except here we use inline
styles to display a bar that is sized proportionate to the number of votes the browser has received. You should now have
a complete poll.

## Summary
In this tutorial we have explored forms, and seen the different approaches to creating and using forms. Whether you
decide to use the [userforms module](http://silverstripe.org/user-forms-module) or create a form in PHP depends on the situation and flexibility
required.
[Next Tutorial >>](4-site-search)<file_sep>/docs/en/topics/page-types.md
# Page Types
## Introduction
Page Types are the basic building blocks of any SilverStripe website. A page type can define:
* The template or templates that are used to display content
* What fields are available to edit in the CMS
* Behaviour specific to a page type – for example a contact form on the ‘Contact Us’ page that sends an email
when the form is submitted
All the pages on the base installation are of the page type "Page". See
[tutorial:2-extending-a-basic-site](/tutorials/2-extending-a-basic-site) for a good introduction to page-types.
## Page type templates
Each page type on your website is a sub-class of the SiteTree class. Usually, you’ll define a class called ‘Page’
and use this template to lay out the basic design elements that don’t change.
Why do we sub-class Page for everything? The easiest way to explain this is to use the example of a search form. If we
create a search form on the Page class, then any other sub-class can also use it in their templates. This saves us
re-defining commonly used forms or controls in every class we use.

Each page type is represented by two classes: a data object and a controller. In the diagrams above and below, the data
objects are black and the controllers are blue. The page controllers are only used when the page type is actually
visited on the website. In our example above, the search form would become a method on the ‘Page_Controller’ class.
Any methods put on the data object will be available wherever we use this page. For example, we put any customizations
we want to do to the CMS for this page type in here.

Page types are created using PHP classes. If you’re not sure about how these work, [click here for a gentler
introduction to PHP classes](http://www-128.ibm.com/developerworks/opensource/library/os-phpobj/).
We put the Page class into a file called Page.php inside `mysite/code`. We also put Page_Controller in here. Any other
classes that are based on Page – for example, the class Page_AnythingElse will also go into Page.php. Likewise, the
StaffPage_Image class will go into StaffPage.php.
## Templates
Take a look at mysite/templates/Page.ss. It contains standard HTML markup, with some differences. We’ll go over
these later, but for now, you can see that this file only generates some of the content – it sets up the
`<html>` tags, deals with the `<head>` section, creates the first-level navigation, and then closes it all off again.
See $Layout? That’s what is doing most of the work when you visit a page. Now take a look at `mysite/templates/Layout/Page.ss`.
This as you can see has a lot more markup in it – it’s what is included into $Layout when the ‘Page’ page type is rendered.
Similarly, `mysite/templates/Layout/HomePage.ss` would be rendered into $Layout when the ‘HomePage’ page type is selected for the
current page you’re viewing.
See the [Page Type Templates](/topics/page-type-templates) page for more information.
## Adding database-fields
Adding database fields is a simple process. You define them in an array of the static variable `$db`, this array is
added on the object class. For example, Page or StaffPage. Every time you run db/build to recompile the manifest, it
checks if any new entries are added to the `$db` array and adds any fields to the database that are missing.
For example, you may want an additional field on a StaffPage class which extends Page, called Author. Author is a
standard text field, and can be [casted](/topics/datamodel) as a variable character object in php (VARCHAR in SQL). In the
following example, our Author field is casted as a variable character object with maximum characters of 50. This is
especially useful if you know how long your source data needs to be.
:::php
class StaffPage extends Page {
static $db = array(
'Author' => 'Varchar(50)'
);
}
class StaffPage_Controller extends Page_Controller {
}
See [datamodel](/topics/datamodel) for a more detailed explanation on adding database fields, and how the SilverStripe data
model works.
## Adding formfields and tabs
See [form](/topics/forms) and [tutorial:2-extending-a-basic-site](/tutorials/2-extending-a-basic-site)
## Removing inherited form fields and tabs
### removeFieldFromTab()
Overloading `getCMSFields()` you can call `removeFieldFromTab()` on a `[api:FieldList]` object. For example, if you don't
want the MenuTitle field to show on your page, which is inherited from `[api:SiteTree]`.
:::php
class StaffPage extends Page {
public function getCMSFields() {
$fields = parent::getCMSFields();
$fields->removeFieldFromTab('Root.Content', 'MenuTitle');
return $fields;
}
}
class StaffPage_Controller extends Page_Controller {
}
### removeByName()
`removeByName()` for normal form fields is useful for breaking inheritance where you know a field in your form isn't
required on a certain page-type.
:::php
class MyForm extends Form {
public function __construct($controller, $name) {
// add a default FieldList of form fields
$member = singleton('Member');
$fields = $member->formFields();
// We don't want the Country field from our default set of fields, so we remove it.
$fields->removeByName('Country');
$actions = new FieldList(
new FormAction('submit', 'Submit')
);
parent::__construct($controller, $name, $fields, $actions);
}
}
This will also work if you want to remove a whole tab e.g. $fields->removeByName('Metadata'); will remove the whole
Metadata tab.
For more information on forms, see [form](/topics/forms), [tutorial:2-extending-a-basic-site](/tutorials/2-extending-a-basic-site)
and [tutorial:3-forms](/tutorials/3-forms).
## Creating a new page:
:::php
$page = new Page();
$page->ParentID = 18; //if you want it to be a child of a certain other page...
$page->Title = "Crazy page";
$page->MetaTitle = "madness";
$page->PageTitle = "Funny";
$page->writeToStage('Stage');
$page->publish('Stage', 'Live');
## Updating a page:
:::php
$page = DataObject::get_one("Page", "ParentID = 18");
$page->Title = "More Serious";
$page->writeToStage('Stage');
$page->Publish('Stage', 'Live');
$page->Status = "Published";
## Deleting pages:
:::php
$pageID = $page->ID;
$stageRecord = Versioned::get_one_by_stage('SiteTree', 'Stage', "SiteTree.ID = $pageID");
if ($stageRecord) $stageRecord->delete();
$liveRecord = Versioned::get_one_by_stage('SiteTree', 'Live', "SiteTree_Live.ID = $pageID");
if ($liveRecord) $liveRecord->delete();
<file_sep>/docs/en/topics/form-validation.md
# Form Validation
Form validation is a combination of PHP and JavaScript
## PHP
### Introduction
Validators are implemented as an argument to the `[api:Form]` constructor. You create a required fields validator like
so. In this case, we're creating a `[api:RequiredFields]` validator - the `[api:Validator]` class itself is an abstract
class.
:::php
public function Form() {
$form = new Form($this, 'Form',
new FieldList(
new TextField('MyRequiredField'),
new TextField('MyOptionalField')
),
new FieldList(
new FormAction('submit', 'Submit')
),
new RequiredFields(array('MyRequiredField'))
);
// Optional: Add a CSS class for custom styling
$form->dataFieldByName('MyRequiredField)->addExtraClass('required');
return $form;
}
### Subclassing Validator
To create your own validator, you need to subclass validator and define two methods:
* **javascript()** Should output a snippet of JavaScript that will get called to perform javascript validation.
* **php($data)** Should return true if the given data is valid, and call $this->validationError() if there were any
errors.
## JavaScript
### Default validator.js implementation
TODO Describe behaviour.js solution easily, how to disable it
Setting fieldEl.requiredErrorMsg or formEl.requiredErrorMsg will override the default error message. Both can include
the string '$FieldLabel', which will be replaced with the field's label. Otherwise, the message is "Please fill out
"$FieldLabel", it is required".
You can use Behaviour to load in the appropriate value:
:::js
Behaviour.register({
'#Form_Form' : {
requiredErrorMsg: "Please complete this question before moving on.",
}
});
### Other validation libraries
By default, SilverStripe forms with an attached Validator instance use the custom Validator.js clientside logic. It is
quite hard to customize, and might not be appropriate for all use-cases. You can disable integrated clientside
validation, and use your own (e.g. [jquery.validate](http://docs.jquery.com/Plugins/Validation)).
Disable for all forms (in `mysite/_config.php`):
:::php
Validator::set_javascript_validation_handler('none');
Disable for a specific form:
:::php
$myForm->getValidator()->setJavascriptValidationHandler('none');
## Related
* Model Validation with [api:DataObject->validate()]<file_sep>/thirdparty/jquery-entwine/spec/spec.entwine.events.js
describe( 'Entwine', function() {
beforeEach(function() {
$('body').append('<div id="dom_test"></div>')
});
afterEach(function() {
$('#dom_test').remove()
});
describe( 'Events', function() {
beforeEach(function() {
$.entwine.synchronous_mode();
$.entwine.clear_all_rules()
$('#dom_test').html('<div id="a" class="a b c"></div>')
});
it( 'calls onfoo when foo triggered', function() {
var a = 0;
$('#a').entwine({onfoo: function(){a = 1;} });
expect(a).toEqual(0);
$('#a').trigger('foo');
expect(a).toEqual( 1);
});
it( 'only calls most specific onfoo when foo triggered', function() {
var a = 0, b = 0;
$('#a.a').entwine({onfoo: function(){a = 1;} });
$('#a').entwine({onfoo: function(){b = 1;} });
expect(a).toEqual( 0);
expect(b).toEqual( 0);
$('#a').trigger('foo');
expect(a).toEqual( 1);
expect(b).toEqual( 0);
});
it( 'calls namespaced onfoo when foo triggered', function() {
var a = 0;
$('#a').entwine('bar', function($){return{onfoo: function(){a = 1;} }});
expect(a).toEqual( 0);
$('#a').trigger('foo');
expect(a).toEqual( 1);
});
it( 'calls most specific namespaced onfoo and most specific non-namespaced onfoo when foo triggered', function() {
var a = 0, b = 0, c = 0, d = 0;
$('#a.a').entwine({onfoo: function(){a = 1;} });
$('#a').entwine({onfoo: function(){b = 1;} });
$('#a.a').entwine('bar', function($){return{onfoo: function(){c = 1;} }});
$('#a').entwine('bar', function($){return{onfoo: function(){d = 1;} }});
expect([a, b, c, d]).toEqual( [0, 0, 0, 0] );
$('#a').trigger('foo');
expect([a, b, c, d]).toEqual( [1, 0, 1, 0]);
});
it( 'calls up correctly on _super', function() {
var a = 0, b = 0;
$('#a').entwine({onfoo: function(){a += 1;} });
$('#a.a').entwine({onfoo: function(){this._super(); b += 1; this._super();} });
expect([a, b]).toEqual( [0, 0]);
$('#a').trigger('foo')
expect([a, b]).toEqual( [2, 1]);
});
it( 'passes event object', function() {
var event;
$('#a').entwine({onfoo: function(e){event = e;} });
$('#a').trigger('foo');
expect(event.type).toBeDefined();
expect(event.type).toEqual('foo');
expect(event.target).toHaveAttr( 'id', 'a');
});
it( 'delegates submit events to forms', function() {
var a = 0;
$('<form class="foo" action="javascript:undefined">').appendTo('#dom_test');
$('.foo').entwine({onsubmit: function(e, d){a = 1;} });
expect(a).toEqual( 0);
$('.foo').trigger('submit');
expect(a).toEqual( 1);
});
describe( 'can pass event data', function() {
it( 'on custom events', function() {
var data;
$('#a').entwine({onfoo: function(e, d){data = d;} });
$('#a').trigger('foo', {cheese: 'burger'});
expect(data.cheese).toEqual( 'burger');
});
it( 'on normal events', function() {
var data;
$('#a').entwine({onclick: function(e, d){data = d;} });
$('#a').trigger('click', {finger: 'left'});
expect(data.finger).toEqual( 'left');
});
it( 'on submit', function() {
var data;
$('<form class="foo" action="javascript:undefined">').appendTo('#dom_test');
$('.foo').entwine({onsubmit: function(e, d){data = d; return false;} })
$('.foo').trigger('submit', {cheese: 'burger'});
expect(data.cheese).toEqual( 'burger');
});
});
describe( 'calls onchange on checkboxes properly', function() {
beforeEach(function() {
$('#dom_test').html('<input id="i" type="checkbox" name="test_input_i" value="i" />');
});
it( 'calls onchange', function() {
var a = 0;
$('#i').entwine({onchange: function(){ a += 1; }});
// Can't just "click()" - it's not the same as an actual click event
$('#i').trigger('focusin'); $('#i')[0].click();
expect(a).toEqual(1);
});
it( 'calls onchange only once per change', function() {
var a = 0;
$('#i').entwine({onchange: function(){ a += 1; }});
$('#i').trigger('focusin'); $('#i')[0].click();
expect(a).toEqual(1);
$('#i').trigger('focusout'); $('#i').trigger('focusin'); $('#i').trigger('focusout');
expect(a).toEqual(1);
$('#i')[0].click();
expect(a).toEqual(2);
});
it( 'calls onchange even if checked attribute altered in mean time', function() {
var a = 0;
$('#i').entwine({onchange: function(){ a += 1; }});
$('#i').trigger('focusin'); $('#i')[0].click();
expect(a).toEqual(1);
$('#i').removeAttr('checked');
$('#i').trigger('focusin'); $('#i')[0].click();
expect(a).toEqual(2);
});
});
});
});<file_sep>/forms/gridfield/GridFieldAddNewButton.php
<?php
/**
* This component provides a button for opening the add new form provided by {@link GridFieldDetailForm}.
*
* @package framework
* @subpackage gridfield
*/
class GridFieldAddNewButton implements GridField_HTMLProvider {
protected $targetFragment;
public function __construct($targetFragment = 'before') {
$this->targetFragment = $targetFragment;
}
public function getHTMLFragments($gridField) {
$data = new ArrayData(array(
'NewLink' => Controller::join_links($gridField->Link('item'), 'new'),
));
return array(
$this->targetFragment => $data->renderWith('GridFieldAddNewbutton'),
);
}
}
<file_sep>/docs/en/howto/grouping-dataobjectsets.md
# Grouping Data Object Sets
The [api:DataObjectSet] class has a number of methods useful for grouping objects by fields. Together with sorting this
can be used to break up long lists of data into more manageable sub-sections.
The [api:DataObjectSet->groupBy()] method takes a field name as the single argument, and breaks the set up into a number
of arrays, where each array contains only objects with the same value of that field. The [api:DataObjectSet->GroupedBy()]
method builds on this and returns the same data in a template-friendly format.
## Grouping Sets By First Letter
This example deals with breaking up a [api:DataObjectSet] into sub-headings by the first letter.
Let's say you have a set of Module objects, each representing a SilverStripe module, and you want to output a list of
these in alphabetical order, with each letter as a heading; something like the following list:
* B
* Blog
* C
* CMS Workflow
* Custom Translations
* D
* Database Plumber
* ...
The first step is to set up the basic data model, along with a method that returns the first letter of the title. This
will be used both for grouping and for the title in the template.
:::php
class Module extends DataObject {
public static $db = array(
'Title' => 'Varchar(255)'
);
// ...
/**
* Returns the first letter of the module title, used for grouping.
*
* @return string
*/
public function getTitleFirstLetter() {
return $this->Title[0];
}
}
The next step is to create a method or variable that will contain/return all the Module objects, sorted by title. For
this example this will be a method on the Page class.
:::php
class Page extends SiteTree {
// ...
/**
* Returns all modules, sorted by their title.
*
* @return DataObjectSet
*/
public function getModules() {
return DataObject::get('Module', null, '"Title"');
}
}
The final step is to render this into a template. The [api:DataObjectSet->GroupedBy()] method breaks up the set into
a number of sets, grouped by the field that is passed as the parameter. In this case, the getTitleFirstLetter method
defined earlier is used to break them up.
:::ss
// Modules list grouped by TitleFirstLetter
<h2>Modules</h2>
<% control Modules.GroupedBy(TitleFirstLetter) %>
<h3>$TitleFirstLetter</h3>
<ul>
<% control Children %>
<li>$Title</li>
<% end_control %>
</ul>
<% end_control %>
## Grouping Sets By Month
Grouping a set by month is a very similar process. The only difference would be to sort the records by month name, and
then create a method on the DataObject that returns the month name, and pass that to the [api:DataObjectSet->GroupedBy()]
call.
Again, the first step is to create a method on the class in question that will be displayed in a list. For this example,
a [api:DataObject] called NewsItem will be used. This will have a method which returns the month it was posted in:
:::php
class NewsItem extends DataObject {
public static $db = array(
'Title' => 'Varchar(255)',
'Date' => 'Date'
);
// ...
/**
* Returns the month name this news item was posted in.
*
* @return string
*/
public function getMonthPosted() {
return date('F', strtotime($this->Date));
}
}
The next step is to create a method that will return all the News records that exist, sorted by month name from
January to December. This can be accomplshed by sorting by the Date field:
:::php
class Page extends SiteTree {
/**
* Returns all news items, sorted by the month they were posted
*
* @return DataObjectSet
*/
public function getNewsItems() {
return DataObject::get('NewsItem', null, '"Date"');
}
}
The final step is the render this into the template using the [api:DataObjectSet->GroupedBy()] method.
:::ss
// Modules list grouped by the Month Posted
<h2>Modules</h2>
<% control NewsItems.GroupedBy(MonthPosted) %>
<h3>$MonthPosted</h3>
<ul>
<% control Children %>
<li>$Title ($Date.Nice)</li>
<% end_control %>
</ul>
<% end_control %> | dd3a14d78930a7e99f1eec49f9b98c61fc60ca66 | [
"Markdown",
"JavaScript",
"PHP"
] | 29 | Markdown | silverstripe-scienceninjas/sapphire | a5355a7f3949c3b5ad69756b7cb08c6295fb63cf | 3ecb121a1c8d44a3f6513f027a92e5621a5c410d |
refs/heads/main | <repo_name>tolgacinar/egegen-project<file_sep>/system/app/libraries/Excel.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
use PhpOffice\PhpSpreadsheet\Spreadsheet;
use PhpOffice\PhpSpreadsheet\Writer\Xlsx;
class Excel {
protected $ci;
protected $spreadsheet;
public function __construct() {
$this->ci =& get_instance();
$this->spreadsheet = new Spreadsheet;
}
public function listToExcel($list, $fields) {
$sheet = $this->spreadsheet->getActiveSheet();
$range = range("A", "Z");
foreach ($list as $key => $value) {
$nkey = 0;
foreach ($value as $k => $v) {
if (in_array($k, $fields)) {
$sheet->setCellValue($range[$nkey] . ($key+1), strip_tags($v));
$nkey++;
}
}
}
$writer = new Xlsx($this->spreadsheet);
$filename = date("Y-m-d-h-i-s");
header('Content-Type: application/vnd.ms-excel');
header('Content-Disposition: attachment;filename="'. $filename .'.xlsx"');
header('Cache-Control: max-age=0');
$writer->save('php://output');
}
}
/* End of file Excel.php */
/* Location: .//D/xampp/htdocs/egegen/system/app/libraries/Excel.php */
<file_sep>/site/controllers/Content.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Content extends MX_Controller {
public function __construct() {
parent::__construct();
$this->load->model('content_model');
}
public function content_detail($url) {
$content = $this->content_model->getContent($url);
if (empty($content)) {
show_404();
}
$this->load->library('breadcrumb');
$this->breadcrumb->add("Yazılar", "#");
$this->breadcrumb->add($content->content_title, $content->s_url);
$data = [
"content" => $content,
"breadcrumb" => $this->breadcrumb->render()
];
$this->layout->title($content->content_title);
$this->layout->render("content_detail", $data);
}
}
<file_sep>/system/app/libraries/Layout.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Layout {
protected $ci;
public $data = [];
public function __construct() {
$this->ci =& get_instance();
$this->title("");
$this->ci->load->model('site/common_model');
$this->data['menus'] = $this->ci->common_model->getMenu();
}
public function render($view, $data = []) {
$data = array_merge($this->data, $data);
$this->ci->load->view("includes/header", $data);
$this->ci->load->view($view);
$this->ci->load->view("includes/footer", $data);
}
public function title($title) {
$this->data['page_title'] = $title . " - Site Adı";
}
}
/* End of file Layout.php */
/* Location: ./application/libraries/Layout.php */
<file_sep>/admin/views/contents/list.php
<div class="row">
<div class="col-12">
<div class="card">
<div class="card-header">
<h3 class="card-title">İçerikler Tablosu</h3>
<div class="card-tools">
<a class="btn btn-sm btn-primary" href="<?php echo base_url("admin/contents/excel") ?>"><i class="fa fa-plus"></i> Excel</a>
<a class="btn btn-sm btn-primary" href="<?php echo base_url("admin/contents/create") ?>"><i class="fa fa-plus"></i> Yeni İçerik</a>
</div>
</div>
<!-- /.card-header -->
<div class="card-body table-responsive p-0">
<table class="table table-hover table-striped text-nowrap">
<thead>
<tr>
<th width="15%">Görsel</th>
<th>Başlık</th>
<th width="15%">İşlem</th>
</tr>
</thead>
<tbody>
<?php foreach ($contents as $key => $content): ?>
<tr>
<td><img src="<?php echo base_url($content->content_image) ?>" style="height:30px;width:auto;"></td>
<td><?php echo $content->content_title; ?></td>
<td>
<a class="btn btn-danger" href="<?php echo base_url("admin/contents/delete/$content->content_id") ?>">Sil</a>
</td>
</tr>
<?php endforeach ?>
</tbody>
</table>
</div>
<!-- /.card-body -->
</div>
<!-- /.card -->
</div>
</div>
<!-- /.row -->
<file_sep>/admin/models/Carousel_model.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Carousel_model extends CI_Model {
public function getSlides() {
return $this->db->get("slides")->result();
}
public function insertCarousel() {
$this->load->library('form_validation');
$this->form_validation->set_rules('slide_title', 'Slide Başlığı', 'trim|required');
if ($_FILES['slide_image']['error'] != 0) {
$this->form_validation->set_rules('slide_image', 'Slide Görseli', 'trim|required');
}
if ($this->form_validation->run() == TRUE) {
$config['upload_path'] = FCPATH . 'uploads/slides/';
$config['allowed_types'] = 'gif|jpg|png';
$config['max_size'] = 2048;
$this->load->library('upload', $config);
if (!$this->upload->do_upload('slide_image')) {
$this->error = $this->upload->display_errors();
return false;
}else {
$insertData = [
"slide_title" => $this->input->post("slide_title", TRUE),
"slide_image" => str_replace(str_replace("\\", "/", FCPATH), "", $this->upload->data("full_path")),
"slide_status" => true
];
if ($this->db->insert("slides", $insertData)) {
return true;
}else {
$this->error = $this->db->error();
}
}
} else {
$this->error = validation_errors();
return false;
}
}
public function deleteCarousel($slide_id) {
$slide = $this->db->get_where("slides", ['slide_id' => $slide_id])->row();
if ($this->db->delete("slides", ['slide_id' => $slide_id])) {
unlink(FCPATH . $slide->slide_image);
return true;
}
}
}
/* End of file Carousel.php */
/* Location: .//D/xampp/htdocs/egegen/admin/models/Carousel.php */<file_sep>/site/models/Content_model.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Content_model extends CI_Model {
public function getContent($url) {
$url = "content/" . $url;
$content = $this->db
->where(["seo_url.s_type" => "content", "seo_url.s_url" => $url])
->join("seo_url", "contents.content_id = seo_url.s_target")
->get("contents")
->row();
return $content;
}
}
/* End of file Content_model.php */
/* Location: ./application/modules/site/models/Content_model.php */<file_sep>/admin/models/News_model.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class News_model extends CI_Model {
public function getNews() {
return $this->db->get("news")->result();
}
public function insertNews() {
$this->load->library('form_validation');
$this->form_validation->set_rules('news_title', 'Haber Başlığı', 'trim|required');
$this->form_validation->set_rules('news_content', 'Haber İçeriği', 'trim|required');
if ($_FILES['news_image']['error'] != 0) {
$this->form_validation->set_rules('news_image', 'Haber Görseli', 'trim|required');
}
if ($this->form_validation->run() == TRUE) {
$config['upload_path'] = FCPATH . 'uploads/news/';
$config['allowed_types'] = 'gif|jpg|png';
$config['max_size'] = 2048;
$this->load->library('upload', $config);
if (!$this->upload->do_upload('news_image')) {
$this->error = $this->upload->display_errors();
return false;
} else {
$insertData = [
"news_title" => $this->input->post("news_title", TRUE),
"news_content" => $this->input->post("news_title", TRUE),
"news_image" => str_replace(str_replace("\\", "/", FCPATH), "", $this->upload->data("full_path")),
"news_status" => true
];
$this->db->trans_begin();
$this->db->insert("news", $insertData);
$news_id = $this->db->insert_id();
$this->db->insert("seo_url", [
"s_type" => "news",
"s_target" => $news_id,
"s_url" => "news/" . permalink($this->input->post("news_title", TRUE))
]);
if ($this->db->trans_status() === FALSE) {
$this->db->trans_rollback();
$this->error = $this->db->error();
return false;
} else {
$this->db->trans_commit();
return true;
}
}
} else {
$this->error = validation_errors();
return false;
}
}
public function deleteNews($news_id) {
$news = $this->db->get_where("news", ['news_id' => $news_id])->row();
$this->db->trans_begin();
$this->db->delete("news", ['news_id' => $news_id]);
$this->db->delete("seo_url", ['s_type' => 'news', 's_target' => $news_id]);
if ($this->db->trans_status() === FALSE) {
$this->db->trans_rollback();
$this->error = $this->db->error();
return false;
} else {
$this->db->trans_commit();
unlink(FCPATH . $news->news_image);
return true;
}
}
}
/* End of file Carousel.php */
/* Location: .//D/xampp/htdocs/egegen/admin/models/Carousel.php */<file_sep>/site/views/home.php
<main>
<section id="hero-area">
<div id="carouselExampleInterval" class="carousel slide" data-bs-ride="carousel">
<div class="carousel-inner">
<?php foreach ($slides as $key => $slide): ?>
<div class="carousel-item <?php echo $key == 0 ? "active" : ""; ?>" data-bs-interval="10000">
<img src="<?php echo $slide->slide_image; ?>" class="d-block w-100" alt="<?php echo $slide->slide_title; ?>">
</div>
<?php endforeach ?>
</div>
<button class="carousel-control-prev" type="button" data-bs-target="#carouselExampleInterval" data-bs-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="visually-hidden">Previous</span>
</button>
<button class="carousel-control-next" type="button" data-bs-target="#carouselExampleInterval" data-bs-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="visually-hidden">Next</span>
</button>
</div>
</section>
<section id="about">
<div class="container">
<div class="row">
<?php foreach ($contents as $content): ?>
<div class="col-12 mt-3 border p-3">
<h2><?php echo $content->content_title; ?></h2>
<p><?php echo $content->content_text; ?></p>
<a href="<?php echo base_url($content->s_url); ?>" class="btn btn-info text-white">Devamı</a>
</div>
<?php endforeach ?>
</div>
</div>
</section>
<section id="contents">
<div class="container">
<div class="row">
<div class="col">
<div class="contents-title d-flex justify-content-between align-items-center">
<h2>Haberler</h2>
<button class="btn more-btn" data-limit="3" data-offset="3"><i class="fas fa-plus"></i></button>
</div>
<div class="contents-list">
<?php foreach ($news as $nws): ?>
<div class="content d-flex mb-3">
<div class="content-img flex-shrink-0">
<img src="<?php echo $nws->news_image; ?>" alt="<?php echo $nws->news_title; ?>">
</div>
<div class="content-text py-2">
<h3 class="content-title"><?php echo $nws->news_title; ?></h3>
<p><?php echo $nws->news_content; ?></p>
</div>
</div>
<?php endforeach ?>
</div>
</div>
</div>
</div>
</section>
</main>
<file_sep>/admin/controllers/Carousel.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Carousel extends CI_Controller {
public function __construct() {
parent::__construct();
$this->load->model('carousel_model', 'carousel');
}
public function index() {
$this->load->model('carousel_model', 'carousel');
$data = [
"slides" => $this->carousel->getSlides(),
];
$this->layout->render("carousel/list", $data);
}
public function create() {
$data = [];
if (isset($_POST) && !empty($_POST)) {
if ($this->carousel->insertCarousel()) {
redirect('admin/carousel','refresh');
}else {
$data['error'] = $this->carousel->error;
}
}
$this->layout->render("carousel/create", $data);
}
public function delete($slide_id) {
if ($this->carousel->deleteCarousel($slide_id)) {
redirect('admin/carousel','refresh');
}
}
}
/* End of file Carousel.php */
/* Location: .//D/xampp/htdocs/egegen/admin/controllers/Carousel.php */<file_sep>/site/controllers/Home.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Home extends MX_Controller {
public function __construct() {
parent::__construct();
$this->load->model('home_model');
}
public function index() {
$data = [
"slides" => $this->home_model->getSlides(),
"contents" => $this->home_model->getContents(),
"news" => $this->home_model->getNews(3)
];
$this->layout->title("Anasayfa");
$this->layout->render("home", $data);
}
public function fetch_news() {
if ($this->input->is_ajax_request() && !empty($_POST)) {
$post = $this->input->post(NULL, TRUE);
$limit = (int) $this->input->post("limit");
$offset = (int) $this->input->post("offset");
$news = $this->home_model->getNews($limit, $offset);
if (empty($news)) {
$this->output->set_content_type('application/json')->set_output(json_encode([
"status" => false
]));
}else {
if ($offset + $limit >= $this->home_model->countNews()) {
$last = true;
}else {
$last = false;
}
$this->output->set_content_type('application/json')->set_output(json_encode([
"status" => true,
"offset" => $offset,
"news" => $this->home_model->getNews($limit, $offset),
"last" => $last
]));
}
}
}
}
<file_sep>/admin/models/Contents_model.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Contents_model extends CI_Model {
public function getContents() {
return $this->db->get("contents")->result();
}
public function insertContent() {
$this->load->library('form_validation');
$this->form_validation->set_rules('content_title', 'İçerik Başlığı', 'trim|required');
$this->form_validation->set_rules('content_text', 'İçerik Metni', 'trim|required');
if ($_FILES['content_image']['error'] != 0) {
$this->form_validation->set_rules('content_image', 'İçerik Görseli', 'trim|required');
}
if ($this->form_validation->run() == TRUE) {
$config['upload_path'] = FCPATH . 'uploads/contents/';
$config['allowed_types'] = 'gif|jpg|png';
$config['max_size'] = 2048;
$this->load->library('upload', $config);
if (!$this->upload->do_upload('content_image')) {
$this->error = $this->upload->display_errors();
return false;
} else {
$insertData = [
"content_title" => $this->input->post("content_title", TRUE),
"content_text" => $this->input->post("content_text", TRUE),
"content_image" => str_replace(str_replace("\\", "/", FCPATH), "", $this->upload->data("full_path")),
"content_status" => true
];
$this->db->trans_begin();
$this->db->insert("contents", $insertData);
$content_id = $this->db->insert_id();
$this->db->insert("seo_url", [
"s_type" => "content",
"s_target" => $content_id,
"s_url" => "content/" . permalink($this->input->post("content_title", TRUE))
]);
if ($this->db->trans_status() === FALSE) {
$this->db->trans_rollback();
$this->error = $this->db->error();
return false;
} else {
$this->db->trans_commit();
return true;
}
}
} else {
$this->error = validation_errors();
return false;
}
}
public function deleteContent($content_id) {
$content = $this->db->get_where("contents", ['content_id' => $content_id])->row();
$this->db->trans_begin();
$this->db->delete("contents", ['content_id' => $content_id]);
$this->db->delete("seo_url", ['s_type' => 'contents', 's_target' => $content_id]);
if ($this->db->trans_status() === FALSE) {
$this->db->trans_rollback();
$this->error = $this->db->error();
return false;
} else {
$this->db->trans_commit();
unlink(FCPATH . $content->content_image);
return true;
}
}
}
/* End of file Contents_model.php */
/* Location: .//D/xampp/htdocs/egegen/admin/models/Contents_model.php */<file_sep>/admin/controllers/Menus.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Menus extends MX_Controller {
public function index() {
$this->layout->render("menu/builder");
}
public function save_menu() {
if ($this->input->post("menu")) {
if($this->db->update("settings", ['set_val' => $this->input->post("menu")], ['set_key' => "menu"])) {
return $this->output->set_content_type('application/json')->set_output(json_encode([
"status" => true,
]));
}else {
return $this->output->set_content_type('application/json')->set_output(json_encode([
"status" => false,
]));
}
}
}
public function get_menu() {
return $this->output->set_content_type('application/json')->set_output($this->db->get_where("settings", ['set_key' => "menu"])->row()->set_val);
}
}
/* End of file Menus.php */
/* Location: ./application/modules/admin/controllers/Menus.php */<file_sep>/egegen.sql
-- phpMyAdmin SQL Dump
-- version 4.8.4
-- https://www.phpmyadmin.net/
--
-- Anamakine: 127.0.0.1
-- Üretim Zamanı: 07 Eki 2021, 11:01:20
-- Sunucu sürümü: 10.4.17-MariaDB
-- PHP Sürümü: 7.0.33
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET AUTOCOMMIT = 0;
START TRANSACTION;
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- Veritabanı: `egegen`
--
-- --------------------------------------------------------
--
-- Tablo için tablo yapısı `contents`
--
CREATE TABLE `contents` (
`content_id` int(11) NOT NULL,
`content_title` varchar(255) NOT NULL,
`content_text` text NOT NULL,
`content_image` varchar(255) NOT NULL,
`content_status` tinyint(1) NOT NULL DEFAULT 0
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Tablo döküm verisi `contents`
--
INSERT INTO `contents` (`content_id`, `content_title`, `content_text`, `content_image`, `content_status`) VALUES
(1, 'İzmir Hakkında', '<p><b>İzmir hakkında</b> texti buraya gelecek</p>', 'uploads/contents/izmir-hakkinda.jpg', 1),
(3, 'qsdqsdqsdqsd', 'azazazaz', 'uploads/contents/okul-toren-bayraklari-8.jpg', 1);
-- --------------------------------------------------------
--
-- Tablo için tablo yapısı `menu`
--
CREATE TABLE `menu` (
`menu_id` int(11) NOT NULL,
`menu_title` varchar(50) NOT NULL,
`menu_href` varchar(100) NOT NULL,
`menu_parent` int(11) NOT NULL,
`menu_status` tinyint(1) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Tablo döküm verisi `menu`
--
INSERT INTO `menu` (`menu_id`, `menu_title`, `menu_href`, `menu_parent`, `menu_status`) VALUES
(1, 'Anasayfa', '/', 0, 1),
(2, 'Yazılar', 'yazilar', 0, 1),
(3, 'Yazı 1', 'yazilar/yazi-1', 2, 1),
(4, 'Yazı 2', 'yazilar/yazi-2', 2, 1),
(5, 'Gezilecek Yerler', 'gezilecek-yerler', 0, 1),
(6, 'Galeri', 'galeri', 0, 1),
(7, 'Galeri Alt 1', 'galeri/galeri-alt-1', 6, 1),
(8, 'Galeri Alt 2', 'galeri/galeri-alt-2', 6, 1),
(9, 'İletişim', 'iletisim', 0, 1);
-- --------------------------------------------------------
--
-- Tablo için tablo yapısı `news`
--
CREATE TABLE `news` (
`news_id` int(11) NOT NULL,
`news_title` varchar(255) NOT NULL,
`news_content` text NOT NULL,
`news_image` varchar(255) NOT NULL,
`news_status` tinyint(1) NOT NULL DEFAULT 0
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Tablo döküm verisi `news`
--
INSERT INTO `news` (`news_id`, `news_title`, `news_content`, `news_image`, `news_status`) VALUES
(1, 'Deneme Haber 1', 'Deneme Haber 1 Texti Buraya Gelecek', 'uploads/news/news-1.jpg', 1),
(2, 'Deneme Haber 2', 'Deneme Haber 2 Texti Buraya Gelecek', 'uploads/news/news-2.jpg', 1),
(3, 'Deneme Haber 3', 'Deneme Haber 3 Texti Buraya Gelecek', 'uploads/news/news-3.jpg', 1),
(4, 'Deneme Haber 4', 'Deneme Haber 4 Texti Buraya Gelecek', 'uploads/news/news-4.jpg', 1),
(5, 'Deneme Haber 5', 'Deneme Haber 5 Texti Buraya Gelecek', 'uploads/news/news-5.jpg', 1),
(6, 'Deneme Haber 6', 'Deneme Haber 6 Texti Buraya Gelecek', 'uploads/news/news-6.jpg', 1),
(7, 'Deneme Haber 7', 'Deneme Haber 7 Texti Buraya Gelecek', 'uploads/news/news-7.jpg', 1),
(8, 'Deneme Haber 8', 'Deneme Haber 8 Texti Buraya Gelecek', 'uploads/news/news-8.jpg', 1),
(9, 'Deneme Haber 9', 'Deneme Haber 9 Texti Buraya Gelecek', 'uploads/news/news-9.jpg', 1),
(10, 'Deneme Haber 10', 'Deneme Haber 10 Texti Buraya Gelecek', 'uploads/news/news-10.jpg', 1),
(14, 'test', 'test', 'uploads/news/ataturk-bayraklari-duvar.jpg', 1);
-- --------------------------------------------------------
--
-- Tablo için tablo yapısı `seo_url`
--
CREATE TABLE `seo_url` (
`s_id` int(11) NOT NULL,
`s_type` varchar(255) NOT NULL,
`s_target` int(11) NOT NULL,
`s_url` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Tablo döküm verisi `seo_url`
--
INSERT INTO `seo_url` (`s_id`, `s_type`, `s_target`, `s_url`) VALUES
(1, 'content', 1, 'content/izmir-hakkinda'),
(2, 'news', 1, 'news/news-1'),
(3, 'news', 2, 'news/news-2'),
(4, 'news', 3, 'news/news-3'),
(5, 'news', 4, 'news/news-4'),
(6, 'news', 5, 'news/news-5'),
(7, 'news', 6, 'news/news-6'),
(8, 'news', 7, 'news/news-7'),
(9, 'news', 8, 'news/news-8'),
(10, 'news', 9, 'news/news-9'),
(11, 'news', 10, 'news/news-10'),
(12, 'news', 12, 'haber-testi'),
(15, 'content', 3, 'content/qsdqsdqsdqsd'),
(16, 'news', 14, 'news/test');
-- --------------------------------------------------------
--
-- Tablo için tablo yapısı `settings`
--
CREATE TABLE `settings` (
`id` int(11) NOT NULL,
`set_key` varchar(255) NOT NULL,
`set_val` longtext NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Tablo döküm verisi `settings`
--
INSERT INTO `settings` (`id`, `set_key`, `set_val`) VALUES
(1, 'menu', '[{\"text\":\"Anasayfa\",\"href\":\"http://localhost\",\"icon\":\"\",\"target\":\"_self\",\"title\":\"\"},{\"text\":\"Yazılar\",\"href\":\"#\",\"icon\":\"empty\",\"target\":\"_self\",\"title\":\"\",\"children\":[{\"text\":\"Yazı 1\",\"href\":\"#\",\"icon\":\"empty\",\"target\":\"_self\",\"title\":\"\"},{\"text\":\"Yazı 2\",\"href\":\"#\",\"icon\":\"empty\",\"target\":\"_self\",\"title\":\"\"}]},{\"text\":\"Gezilecek Yerler\",\"href\":\"#\",\"icon\":\"empty\",\"target\":\"_self\",\"title\":\"\"},{\"text\":\"Galeri\",\"href\":\"#\",\"icon\":\"empty\",\"target\":\"_self\",\"title\":\"\",\"children\":[{\"text\":\"Galeri 1\",\"href\":\"#\",\"icon\":\"empty\",\"target\":\"_self\",\"title\":\"\"},{\"text\":\"Galeri 2\",\"href\":\"#\",\"icon\":\"empty\",\"target\":\"_self\",\"title\":\"\"}]},{\"text\":\"İletişim\",\"href\":\"#\",\"icon\":\"empty\",\"target\":\"_self\",\"title\":\"\"}]');
-- --------------------------------------------------------
--
-- Tablo için tablo yapısı `slides`
--
CREATE TABLE `slides` (
`slide_id` int(11) NOT NULL,
`slide_title` varchar(255) NOT NULL,
`slide_image` varchar(255) NOT NULL,
`slide_status` tinyint(1) NOT NULL DEFAULT 0
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Tablo döküm verisi `slides`
--
INSERT INTO `slides` (`slide_id`, `slide_title`, `slide_image`, `slide_status`) VALUES
(1, 'Slide 1', 'uploads/slides/slide-1.jpg', 1),
(2, 'Slide 2', 'uploads/slides/slide-2.jpg', 1),
(3, 'Slide 3', 'uploads/slides/slide-3.jpg', 1),
(9, 'test', 'uploads/slides/ataturk-bayraklari-duvar-5.jpg', 1);
--
-- Dökümü yapılmış tablolar için indeksler
--
--
-- Tablo için indeksler `contents`
--
ALTER TABLE `contents`
ADD PRIMARY KEY (`content_id`);
--
-- Tablo için indeksler `menu`
--
ALTER TABLE `menu`
ADD PRIMARY KEY (`menu_id`);
--
-- Tablo için indeksler `news`
--
ALTER TABLE `news`
ADD PRIMARY KEY (`news_id`);
--
-- Tablo için indeksler `seo_url`
--
ALTER TABLE `seo_url`
ADD PRIMARY KEY (`s_id`);
--
-- Tablo için indeksler `settings`
--
ALTER TABLE `settings`
ADD PRIMARY KEY (`id`);
--
-- Tablo için indeksler `slides`
--
ALTER TABLE `slides`
ADD PRIMARY KEY (`slide_id`);
--
-- Dökümü yapılmış tablolar için AUTO_INCREMENT değeri
--
--
-- Tablo için AUTO_INCREMENT değeri `contents`
--
ALTER TABLE `contents`
MODIFY `content_id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=4;
--
-- Tablo için AUTO_INCREMENT değeri `menu`
--
ALTER TABLE `menu`
MODIFY `menu_id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=10;
--
-- Tablo için AUTO_INCREMENT değeri `news`
--
ALTER TABLE `news`
MODIFY `news_id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=15;
--
-- Tablo için AUTO_INCREMENT değeri `seo_url`
--
ALTER TABLE `seo_url`
MODIFY `s_id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=17;
--
-- Tablo için AUTO_INCREMENT değeri `settings`
--
ALTER TABLE `settings`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=2;
--
-- Tablo için AUTO_INCREMENT değeri `slides`
--
ALTER TABLE `slides`
MODIFY `slide_id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=10;
COMMIT;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
<file_sep>/site/views/content_detail.php
<main>
<section id="breadcrumbs">
<div class="container-fluid">
<div class="row">
<div class="col">
<nav aria-label="breadcrumb">
<?php echo $breadcrumb ?>
</nav>
</div>
</div>
</div>
</section>
<section id="content-area">
<div class="container">
<div class="row">
<div class="col">
<div class="content-image">
<img src="<?php echo base_url($content->content_image); ?>" alt="<?php echo $content->content_title; ?>">
</div>
<h1><?php echo $content->content_title ?></h1>
<?php echo $content->content_text; ?>
</div>
</div>
</div>
</section>
</main><file_sep>/site/models/Home_model.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Home_model extends CI_Model {
public function __construct() {
parent::__construct();
$this->load->database();
}
public function getSlides() {
return $this->db->where('slide_status', 1)->get("slides")->result();
}
public function getContents() {
return $this->db
->select("contents.*, seo_url.s_url")
->where(["contents.content_status" => 1, "seo_url.s_type" => "content"])
->join("seo_url", "contents.content_id = seo_url.s_target", "left")
->get("contents")
->result();
}
public function getNews($limit = 0, $offset = 0) {
return $this->db
->order_by("news.news_id", "desc")
->where(["news.news_status" => 1, "seo_url.s_type" => "news"])
->limit($limit, $offset)
->join("seo_url", "news.news_id = seo_url.s_target", "left")
->get("news")
->result();
}
public function countNews() {
return $this->db->get_where("news", ["news_status" => 1])->num_rows();
}
}
/* End of file Home_model.php */
/* Location: ./application/modules/site/models/Home_model.php */<file_sep>/site/models/Common_model.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Common_model extends CI_Model {
public function __construct() {
parent::__construct();
$this->load->database();
}
public function getMenu() {
return json_decode($this->db->get_where("settings", ['set_key' => 'menu'])->row()->set_val);
// return $this->buildTree($this->db->where("menu_status", 1)->get("menu")->result());
}
public function buildTree($elements, $parent = 0) {
$return = [];
foreach ($elements as $element) {
if ($element->menu_parent == $parent) {
$children = $this->buildTree($elements, $element->menu_id);
if ($children) {
$element->children = $children;
}else {
$element->children = null;
}
$return[] = $element;
}
}
return $return;
}
public function getSlides() {
return $this->db->where('slide_status', 1)->get("slides")->result();
}
}
/* End of file Common.php */
/* Location: ./application/modules/site/models/Common.php */<file_sep>/admin/controllers/News.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class News extends CI_Controller {
public function __construct() {
parent::__construct();
$this->load->model('news_model', 'news');
}
public function index() {
$data = [
"news" => $this->news->getNews(),
];
$this->layout->render("news/list", $data);
}
public function create() {
$data = [];
if (isset($_POST) && !empty($_POST)) {
if ($this->news->insertNews()) {
redirect('admin/news','refresh');
}else {
$data['error'] = $this->news->error;
}
}
$this->layout->render("news/create", $data);
}
public function delete($news_id) {
if ($this->news->deleteNews($news_id)) {
redirect('admin/news','refresh');
}
}
}
/* End of file News.php */
/* Location: .//D/xampp/htdocs/egegen/admin/controllers/News.php */<file_sep>/README.md
### Kurulum
- Dosyaları çıkardıktan sonra ana dizinde "composer install" komutu kullanılmalıdır.
- Veritabanını içeri aktarmayı unutmayın.
<file_sep>/site/views/includes/header.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title><?php echo $page_title; ?></title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.0/dist/css/bootstrap.min.css" rel="stylesheet" integrity="<KEY>" crossorigin="anonymous">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.4/css/all.min.css" integrity="<KEY> crossorigin="anonymous" referrerpolicy="no-referrer" />
<style>
/* logo ayarı */
.header .navbar-brand {
margin-right: 50px;
}
#hero-area {
width: 100%;
}
/* Menü */
/* ============ desktop view ============ */
@media all and (min-width: 992px) {
.dropdown-menu li{
position: relative;
}
.dropdown-menu .submenu{
display: none;
position: absolute;
left:100%; top:-7px;
}
.dropdown-menu .submenu-left{
right:100%; left:auto;
}
.dropdown-menu > li:hover{ background-color: #f1f1f1 }
.dropdown-menu > li:hover > .submenu{
display: block;
}
}
/* ============ desktop view .end// ============ */
/* ============ small devices ============ */
@media (max-width: 991px) {
.dropdown-menu .dropdown-menu{
margin-left:0.7rem; margin-right:0.7rem; margin-bottom: .5rem;
}
}
/* ============ small devices .end// ============ */
/* Genel Boyutlar */
section h2 {
font-size: 1.6rem;
}
h3 {
font-size: 1.3rem;
}
section:not(:first-child) {
padding: 40px 0px;
}
/* Hakkımızda Bölümü */
/* Anasayfa slider ayarları */
.carousel-item {
height: 600px;
}
.carousel-item img {
object-fit: cover;
height: 100%;
width: 100%;
}
/* Content Stilleri */
.content {
gap: 20px;
}
.content-img {
width: 300px;
height: 200px;
}
.content-img img {
object-fit: cover;
display: block;
width: 100%;
height: 100%;
}
/* Content Alanı */
section#breadcrumbs {
padding: 0;
background-color: #ddd;
}
section#breadcrumbs .breadcrumb {
margin-top: 1rem;
}
section#content-area .content-image {
width: 100%;
height: 500px;
margin-bottom: 40px;
}
section#content-area .content-image img {
width: 100%;
height: 100%;
object-fit: cover;
}
/* Footer */
footer.footer {
height: 50px;
}
</style>
</head>
<body>
<header class="header">
<nav class="navbar navbar-expand-lg navbar-light bg-white">
<div class="container-fluid">
<a class="navbar-brand" href="<?php echo base_url(); ?>">Logo</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#main_nav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="main_nav">
<ul class="navbar-nav">
<?php foreach ($menus as $menu): ?>
<li class="nav-item <?php echo isset($menu->children) ? "dropdown" : ""; ?>">
<a class="nav-link <?php echo isset($menu->children) ? "dropdown-toggle" : ""; ?>" <?php echo isset($menu->children) ? "data-bs-toggle=\"dropdown\"" : ""; ?> href="<?php echo base_url($menu->href); ?>"><?php echo $menu->text; ?></a>
<?php if (isset($menu->children)): ?>
<ul class="dropdown-menu">
<?php foreach ($menu->children as $child): ?>
<li><a class="dropdown-item" href="<?php echo $child->href; ?>"><?php echo $child->text; ?></a></li>
<?php endforeach ?>
</ul>
<?php endif ?>
</li>
<?php endforeach ?>
</ul>
</div> <!-- navbar-collapse.// -->
</div> <!-- container-fluid.// -->
</nav>
</header><file_sep>/admin/controllers/Admin.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Admin extends MX_Controller {
public function index() {
$this->layout->render("main");
}
}
/* End of file Admin.php */
/* Location: ./application/modules/admin/controllers/Admin.php */<file_sep>/admin/views/includes/footer.php
</div><!-- /.container-fluid -->
</div>
<!-- /.content -->
</div>
<!-- /.content-wrapper -->
<!-- Control Sidebar -->
<aside class="control-sidebar control-sidebar-dark">
<!-- Control sidebar content goes here -->
<div class="p-3">
<h5>Title</h5>
<p>Sidebar content</p>
</div>
</aside>
<!-- /.control-sidebar -->
<!-- Main Footer -->
<footer class="main-footer">
<!-- To the right -->
<div class="float-right d-none d-sm-inline">
Anything you want
</div>
<!-- Default to the left -->
<strong>Copyright © 2014-2021 <a href="https://adminlte.io">AdminLTE.io</a>.</strong> All rights reserved.
</footer>
</div>
<!-- ./wrapper -->
<!-- REQUIRED SCRIPTS -->
<!-- jQuery -->
<script src="<?php echo base_url("assets/admin/plugins/jquery/jquery.min.js") ?>"></script>
<!-- Bootstrap 4 -->
<script src="<?php echo base_url("assets/admin/plugins/bootstrap/js/bootstrap.bundle.min.js") ?>"></script>
<script src="<?php echo base_url("assets/admin/plugins/bs-custom-file-input/bs-custom-file-input.min.js") ?>"></script>
<script type="text/javascript" src="<?php echo base_url("assets/admin/dist/js/jquery-menu-editor.min.js") ?>"></script>
<script type="text/javascript" src="<?php echo base_url("assets/admin/plugins/bootstrap-iconpicker/js/iconset/fontawesome5-3-1.min.js") ?>"></script>
<script type="text/javascript" src="<?php echo base_url("assets/admin/plugins/bootstrap-iconpicker/js/bootstrap-iconpicker.min.js") ?>"></script>
<script type="text/javascript" src="<?php echo base_url("assets/admin/plugins/toastr/toastr.min.js") ?>"></script>
<!-- AdminLTE App -->
<script src="<?php echo base_url("assets/admin/dist/js/adminlte.min.js") ?>"></script>
<script type="text/javascript">
$(function () {
bsCustomFileInput.init();
});
$(document).ready(function() {
if($("#menuBuilder").length) {
var iconPickerOptions = {searchText: "Ara...", labelHeader: "{0}/{1}"};
var sortableListOptions = {
placeholderCss: {'background-color': "#cccccc"}
};
var editor = new MenuEditor('myEditor', {listOptions: sortableListOptions, iconPicker: iconPickerOptions});
editor.setForm($('#frmEdit'));
editor.setUpdateButton($('#btnUpdate'));
$.get('/admin/menus/get_menu', function(data) {
editor.setData(data);
});
$('#btnSave').on('click', function (e) {
var str = editor.getString();
e.preventDefault();
$.ajax({
type: "POST",
data: {menu:str},
url: "/admin/menus/save_menu",
success: function(donen) {
if (donen.status) {
toastr.success("Menü başarıyla kaydedildi.", "Menü")
}else {
toastr.error("Menü kaydedilirken bir hata meydana geldi.", "Menü")
}
}
});
});
$("#btnUpdate").click(function(){
editor.update();
});
$('#btnAdd').click(function(){
editor.add();
});
$('[data-toggle="tooltip"]').tooltip();
}
});
</script>
</body>
</html>
<file_sep>/admin/views/contents/create.php
<div class="row">
<div class="col-lg-12">
<div class="card card-info">
<div class="card-header">
<h3 class="card-title">Horizontal Form</h3>
</div>
<!-- /.card-header -->
<!-- form start -->
<form class="form-horizontal" method="post" action="<?php echo base_url("admin/contents/create") ?>" enctype="multipart/form-data">
<div class="card-body">
<?php if (isset($error)): ?>
<div class="form-group alert alert-danger">
<?php echo $error; ?>
</div>
<?php endif ?>
<div class="form-group row">
<label for="inputEmail3" class="col-sm-2 col-form-label">İçerik Başlığı</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="inputEmail3" name="content_title">
</div>
</div>
<div class="form-group row">
<label for="inputPassword3" class="col-sm-2 col-form-label">İçerik Görseli</label>
<div class="col-sm-10">
<div class="custom-file">
<input type="file" class="custom-file-input" name="content_image" id="customFile">
<label class="custom-file-label" for="customFile">Görsel seçin</label>
</div>
</div>
</div>
<div class="form-group row">
<label for="inputEmail3" class="col-sm-2 col-form-label">İçerik Metni</label>
<div class="col-sm-10">
<textarea class="form-control" name="content_text"></textarea>
</div>
</div>
</div>
<!-- /.card-body -->
<div class="card-footer">
<button type="submit" class="btn btn-info">Kaydet</button>
</div>
<!-- /.card-footer -->
</form>
</div>
</div>
</div><file_sep>/admin/controllers/Contents.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Contents extends CI_Controller {
public function __construct() {
parent::__construct();
$this->load->model('contents_model', 'contents');
}
public function index() {
$data = [
"contents" => $this->contents->getContents(),
];
$this->layout->render("contents/list", $data);
}
public function create() {
$data = [];
if (isset($_POST) && !empty($_POST)) {
if ($this->contents->insertContent()) {
redirect('admin/contents','refresh');
}else {
$data['error'] = $this->contents->error;
}
}
$this->layout->render("contents/create", $data);
}
public function delete($content_id) {
if ($this->contents->deleteContent($content_id)) {
redirect('admin/contents','refresh');
}
}
public function excel() {
$this->load->library('excel');
$this->excel->listToExcel($this->contents->getContents(), ["content_title", "content_text", "content_image"]);
}
}
/* End of file Contents.php */
/* Location: .//D/xampp/htdocs/egegen/admin/controllers/Contents.php */ | 6824965e5fef84db8bce240ef12b31cb55fb646a | [
"Markdown",
"SQL",
"PHP"
] | 23 | PHP | tolgacinar/egegen-project | dbfb1cc20221f5318a668b9057d5c3869d83c8a6 | 2c92051ba443fddf170f0a579bb48af936003d58 |
refs/heads/master | <file_sep>require 'pry'
# Create a checkout method to calculate the total cost of a cart of items and apply discounts and coupons as necessary.
cart = [
{"AVOCADO" => {:price => 3.00, :clearance => true}},
{"KALE" => {:price => 3.00, :clearance => false}},
{"BLACK_BEANS" => {:price => 2.50, :clearance => false}},
{"ALMONDS" => {:price => 9.00, :clearance => false}},
{"TEMPEH" => {:price => 3.00, :clearance => true}},
{"CHEESE" => {:price => 6.50, :clearance => false}},
{"BEER" => {:price => 13.00, :clearance => false}},
{"PEANUTBUTTER" => {:price => 3.00, :clearance => true}},
{"BEETS" => {:price => 2.50, :clearance => false}},
{"SOY MILK" => {:price => 4.50, :clearance => true}}
]
coupons = [
{:item => "AVOCADO", :num => 2, :cost => 5.00},
{:item => "BEER", :num => 2, :cost => 20.00},
{:item => "CHEESE", :num => 3, :cost => 15.00}
]
# The cart starts as an array of individual items.
# Translate it into a hash that includes the counts for each item with the consolidate_cart method.
def consolidate_cart(cart)
result = {}
cart.each do |items| # [{"AVOCADO" =>{..}]
items.each do |item, data|
count = cart.count(items) #count = counting each item in cart
data[:count] = count #create key :count that will keep track of number of items
result[item] ||= {} # true if key has value, else value is empty {}
result[item] = data #put it all together
end
end
result
end
# apply coupon to the cart,
# adds a new key, value pair to the cart hash called 'ITEM NAME W/COUPON'
# adds the coupon price to the property hash of couponed item
# adds the count number to the property hash of couponed item
# removes the number of discounted items from the original item's count
# remembers if the item was on clearance
# accounts for when there are more items than the coupon allows
# doesn't break if the coupon doesn't apply to any items
def apply_coupons(cart, coupons)
cart = cart.dup
coupons.each do |coupon|
item = coupon[:item]
#cart[item]=if item in cart matches coupon item
if cart[item] && (cart[item][:count] >=coupon[:num])#amount in cart >= coupon limit
cart[item][:count] -= coupon[:num] #remove discount items from cart
unless cart["#{item} W/COUPON"] # create a coupon if none exists, this {}:
cart["#{item} W/COUPON"] = {:price => coupon[:cost],
:clearance => cart[item][:clearance],
:count => 1}
else
cart["#{item} W/COUPON"][:count] += 1 #increase :count if num items > coupon limit
end
end
end
cart
end
# apply clearance:
# takes 20% off price if the item is on clearance
# does not discount the price for items not on clearance
def apply_clearance(cart)
cart = cart.dup
cart.each do |item, data|
# if clearance is true = take 20% off; no discount = return cart
data[:clearance] ? data[:price] = (data[:price]*0.8).round(2) : cart
end
end
#{}`checkout` method that calculates the total cost of the consolidated cart.
#When checking out, follow these steps *in order*:
#* Apply coupon discounts if the proper number of items are present.
#* Apply 20% discount if items are on clearance.
#* If, after applying the coupon discounts and the clearance discounts, the cart's total is over $100, then apply a 10% discount.
def checkout(cart, coupons)
#call methods and track discounts
cart = consolidate_cart(cart)
cart = apply_coupons(cart, coupons)
cart = apply_clearance(cart)
total = 0
cart.each { |item, data| total += (data[:price] * data[:count]).to_f }
total > 100 ? (total *= 0.9).round(2) : total
end
| ede69f6ecfed301b01e3f6278e45e0f9729e6670 | [
"Ruby"
] | 1 | Ruby | magic8baller/green_grocer-prework | db18ffbbe068073a5fd6a36ecdc7bb0754cf313d | 8f6c31d1539691dd8b458022a889e9b581cbc221 |
refs/heads/master | <file_sep>package com.example.tttkotlin.activity
import android.content.Intent
import android.os.Bundle
import android.text.method.HideReturnsTransformationMethod
import android.text.method.PasswordTransformationMethod
import android.view.animation.AnimationUtils
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
import com.example.tttkotlin.R
import com.example.tttkotlin.api.RetrofitInstance
import com.example.tttkotlin.model.Status
import kotlinx.android.synthetic.main.activity_dang_ky_t_k.*
import retrofit2.Call
import retrofit2.Callback
import retrofit2.Response
import java.util.regex.Pattern
class DangKyTKActivity : AppCompatActivity(){
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_dang_ky_t_k)
val animation = AnimationUtils.loadAnimation(this, R.anim.uptodowndiagonal)
rLayoutDK.setAnimation(animation)
val animationx = AnimationUtils.loadAnimation(this, R.anim.tranlation_x)
tvNhapEmail.setAnimation(animationx)
edtNhapEmailDK.setAnimation(animationx)
tvNhapUserName.setAnimation(animationx)
edtNhapUsernameDK.setAnimation(animationx)
tvNhapPass1.setAnimation(animationx)
edtNhapPass1.setAnimation(animationx)
tvNhapPass2.setAnimation(animationx)
edtNhapPass2.setAnimation(animationx)
checkShowPassDK.setAnimation(animationx)
checkShowPassDK.setOnClickListener{
if(checkShowPassDK.isChecked) {
edtNhapPass1.transformationMethod = HideReturnsTransformationMethod.getInstance()
edtNhapPass2.transformationMethod = HideReturnsTransformationMethod.getInstance()
} else {
edtNhapPass1.transformationMethod = PasswordTransformationMethod.getInstance()
edtNhapPass2.transformationMethod = PasswordTransformationMethod.getInstance()
}
}
cvDKTK.setOnClickListener {
val username = edtNhapUsernameDK.text.toString().trim()
val email = edtNhapEmailDK.text.toString().trim()
val pass1 = edtNhapPass1.text.toString().trim()
val pass2 = edtNhapPass2.text.toString().trim()
if(username.isEmpty()){
edtNhapUsernameDK.error = "Vui lòng kiểm tra lại tên người dùng!"
edtNhapUsernameDK.requestFocus()
return@setOnClickListener
}
if(email.isEmpty()){
edtNhapEmailDK.error = "Vui lòng kiểm tra lại email!"
edtNhapEmailDK.requestFocus()
return@setOnClickListener
}
if(!checkFormatEmail(email)){
edtNhapEmailDK.error = "Email không hợp lệ! Ví dụ hợp lệ: <EMAIL>"
edtNhapEmailDK.requestFocus()
return@setOnClickListener
}
if(pass1.isEmpty()){
edtNhapPass1.error = "Vui lòng kiểm tra lại mật khẩu!"
edtNhapPass1.requestFocus()
return@setOnClickListener
}
if(!checkFormatPass(pass1)){
edtNhapPass1.error = "Mật khẩu phải ít nhất 8 ký tự bao gồm chữ, ký tự đặc biệt và số!"
edtNhapPass1.requestFocus()
return@setOnClickListener
}
if(pass2.isEmpty()){
edtNhapPass2.error = "Vui lòng kiểm tra lại mật khẩu!"
edtNhapPass2.requestFocus()
return@setOnClickListener
}
if(!pass1.equals(pass2) || !pass2.equals(pass1))
edtNhapPass2.error="Mật khẩu chưa trùng khớp!"
else dangKyTaiKhoan(username, email, pass1)
}
}
fun dangKyTaiKhoan(username: String, email: String, pass: String){
RetrofitInstance.instance.dangKyTaiKhoan(username, email, pass).enqueue(object : Callback<Status> {
override fun onResponse(call: Call<Status>, response: Response<Status>) {
response.body()?.let {
if (it.getStatus() == 0) {
Toast.makeText(applicationContext, "Đăng ký tài khoản thành công!", Toast.LENGTH_SHORT).show()
val intent = Intent(applicationContext, LoginActivity::class.java)
startActivity(intent)
onBackPressed()
}
if (it.getStatus() == 1) {
edtNhapUsernameDK.error = "Vui lòng kiểm tra lại tên người dùng!"
}
if (it.getStatus() == 2) {
edtNhapUsernameDK.error = "Vui lòng kiểm tra lại email!"
}
if (it.getStatus() == 3) {
edtNhapPass1.error = "Vui lòng kiểm tra lại mật khẩu!"
edtNhapPass2.error = "Vui lòng kiểm tra lại mật khẩu!"
}
if (it.getStatus() == 4) {
edtNhapEmailDK.error = "Email đã tồn tại!"
}
}
}
override fun onFailure(call: Call<Status>, t: Throwable) {
Toast.makeText(applicationContext, "Lỗi!" + t.message, Toast.LENGTH_SHORT).show()
}
})
}
fun checkFormatEmail(email: String): Boolean {
val EMAIL_ADDRESS_PATTERN = Pattern.compile(
"[a-zA-Z0-9\\+\\.\\_\\%\\-\\+]{1,256}" +
"\\@" +
"[a-zA-Z0-9][a-zA-Z0-9\\-]{0,64}" +
"(" +
"\\." +
"[a-zA-Z0-9][a-zA-Z0-9\\-]{0,25}" +
")+"
)
return EMAIL_ADDRESS_PATTERN.matcher(email).matches()
// val expression = "[a-zA-Z0-9._-]+@[a-z]+\\\\.+[a-z]+"
// val pattern: Pattern = Pattern.compile(expression, Pattern.CASE_INSENSITIVE)
// val matcher: Matcher = pattern.matcher(email)
// Toast.makeText(applicationContext, matcher.matches().toString(), Toast.LENGTH_SHORT).show()
// return matcher.matches()
}
fun checkFormatPass(pass: String): Boolean {
val PASS_PATTERN = Pattern.compile(
"^(?=.*[0-9])"
+ "(?=.*[a-z])(?=.*[A-Z])"
+ "(?=.*[@#$%^&+=])"
+ "(?=\\S+$).{8,20}$"
)
return PASS_PATTERN.matcher(pass).matches()
}
}<file_sep>package com.example.tttkotlin.fragment
import android.content.Intent
import android.os.Bundle
import androidx.fragment.app.Fragment
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.ListView
import com.example.tttkotlin.R
import com.example.tttkotlin.activity.BodyActivity
import com.example.tttkotlin.model.MauTin
import com.example.tttkotlin.adapter.MauTinAdapter
import com.example.tttkotlin.api.RetrofitInstance
import kotlinx.android.synthetic.main.fragment_boi_loi.view.*
import kotlinx.android.synthetic.main.fragment_bong_chuyen.view.*
import kotlinx.android.synthetic.main.fragment_bong_chuyen.view.shimmerFrame
import retrofit2.Call
import retrofit2.Callback
import retrofit2.Response
class BoiLoiFragment : Fragment() {
lateinit var listMauTin:ArrayList<MauTin>
lateinit var viewBoiLoiFragment: View
override fun onCreateView(
inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?): View? {
viewBoiLoiFragment = inflater.inflate(R.layout.fragment_boi_loi, container, false)
listMauTin = arrayListOf()
loadListView()
return viewBoiLoiFragment
}
override fun onResume() {
super.onResume()
viewBoiLoiFragment.shimmerFrame.startShimmer()
}
fun loadListView(){
RetrofitInstance.instance.getListMauTin3().enqueue(object : Callback<ArrayList<MauTin>>{
override fun onResponse(call: Call<ArrayList<MauTin>>, response: Response<ArrayList<MauTin>>) {
response.body()?.let {
listMauTin.addAll(it)
val adapterMauTin = MauTinAdapter(requireContext(), listMauTin)
viewBoiLoiFragment.apply {
listViewMauTinBL.apply {
adapter = adapterMauTin
setOnItemClickListener { parent, view, position, id ->
showChiTietPost(listMauTin.get(position).getIdMauTin().toString())
}
}
shimmerFrame.apply {
stopShimmer()
visibility = View.GONE
}
}
}
}
override fun onFailure(call: Call<ArrayList<MauTin>>, t: Throwable) {
}
})
}
private fun showChiTietPost(id: String?) {
val intent = Intent(context, BodyActivity::class.java)
intent.putExtra("id_post", id)
requireActivity().startActivity(intent)
}
}<file_sep>package com.example.tttkotlin.activity
import android.content.Context
import android.content.Intent
import android.content.SharedPreferences
import android.os.Bundle
import android.view.View
import android.view.animation.AnimationUtils
import android.widget.*
import androidx.appcompat.app.AppCompatActivity
import com.example.tttkotlin.R
import com.example.tttkotlin.SearchActivity
import com.example.tttkotlin.adapter.MauTinAdapter
import com.example.tttkotlin.adapter.TabAdapter
import com.example.tttkotlin.fragment.BoiLoiFragment
import com.example.tttkotlin.fragment.BongChuyenFragment
import com.example.tttkotlin.fragment.BongDaFragment
import com.example.tttkotlin.model.DanhMuc
import com.example.tttkotlin.model.MauTin
import com.google.android.material.navigation.NavigationView
import com.google.android.material.tabs.TabLayout
import com.google.android.material.tabs.TabLayoutMediator
import kotlinx.android.synthetic.main.activity_login_new.*
import kotlinx.android.synthetic.main.activity_main.*
import kotlinx.android.synthetic.main.nav_header.*
import java.util.*
import kotlin.collections.ArrayList
class MainActivity : AppCompatActivity(){
lateinit var listDanhMuc : ArrayList<DanhMuc>
lateinit var tabLayout : TabLayout
lateinit var listMauTin:ArrayList<MauTin>
lateinit var searchView: SearchView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
//replaceFragment(HomeFragment())
tabLayout = findViewById(R.id.tabLayout)
listDanhMuc = arrayListOf()
loadTabLayout()
val sharedPreferences: SharedPreferences = getSharedPreferences("dataLogin", Context.MODE_PRIVATE)
val navigationView = findViewById(R.id.nav_view) as NavigationView
val headerView = navigationView.getHeaderView(0)
val navUsername:TextView = headerView.findViewById<View>(R.id.tvHoTenNa_Header) as TextView
val btnLoginHeader:Button = headerView.findViewById<View>(R.id.btnHeaderLogin) as Button
val imgAVTHeader:ImageView = headerView.findViewById<View>(R.id.imgAVT) as ImageView
var check = sharedPreferences.getString("token", "-1")
if(!check.equals("-1")){//đã đăng nhập
//onBackPressed()
navUsername.setText(sharedPreferences.getString("username", "username"))
btnLoginHeader.setText("Đăng xuất")
imgAVTHeader.setImageResource(R.drawable.deappool)
btnLoginHeader.setOnClickListener {
val intent = Intent(applicationContext, MainActivity::class.java)
startActivity(intent)
var editor = sharedPreferences.edit()
editor.apply {
editor.remove("email")
editor.remove("pass")
editor.remove("token")
editor.remove("username")
}.apply()
editor.commit()
Toast.makeText(applicationContext, "Đăng xuất thành công!", Toast.LENGTH_SHORT).show()
}
}
if(check.equals("-1")){//chưa đăng nhập
navUsername.setText("username");
btnLoginHeader.setText("Đăng nhập")
imgAVTHeader.setImageResource(R.drawable.ic_launcher_foreground)
btnLoginHeader.setOnClickListener {
val intent = Intent(applicationContext, LoginActivity::class.java)
startActivity(intent)
}
}
search()
iconToolbar.setOnClickListener(View.OnClickListener {
drawerlayout.toggleMenu()
})
nav_view.setNavigationItemSelectedListener {
when (it.itemId) {
R.id.H -> {
Toast.makeText(applicationContext, "Home!", Toast.LENGTH_SHORT).show()
true
}
R.id.CS -> {
Toast.makeText(applicationContext, "Chia sẻ!", Toast.LENGTH_SHORT).show()
true
}
R.id.GY -> {
Toast.makeText(applicationContext, "Góp ý!", Toast.LENGTH_SHORT).show()
true
}
R.id.CD -> {
Toast.makeText(applicationContext, "Cài đặt!", Toast.LENGTH_SHORT).show()
true
}
R.id.HT -> {
Toast.makeText(applicationContext, "Hỗ trợ!", Toast.LENGTH_SHORT).show()
true
}
R.id.DGUD -> {
Toast.makeText(applicationContext, "Đánh giá ứng dụng!", Toast.LENGTH_SHORT).show()
true
}
R.id.DKSD -> {
Toast.makeText(applicationContext, "Điều khoản sử dụng!", Toast.LENGTH_SHORT).show()
true
}
else -> false
}
}
}
fun search(){
searchView = findViewById(R.id.searchView)
searchView.setOnQueryTextListener(object : SearchView.OnQueryTextListener {
override fun onQueryTextSubmit(query: String): Boolean {
val intent = Intent(applicationContext, SearchActivity::class.java)
intent.putExtra("textSearch", query)
startActivity(intent)
return false
}
override fun onQueryTextChange(newText: String): Boolean {
return false
}
})
}
fun loadTabLayout(){
val tabadapter = TabAdapter(this@MainActivity, mutableListOf(BongChuyenFragment(), BoiLoiFragment(), BongDaFragment()))
viewPager.adapter = tabadapter
TabLayoutMediator(tabLayout,viewPager,{ tab,pos->
when(pos){
0-> tab.text = "Bóng đá"
1->tab.text="Bơi lội"
2->tab.text="Bóng bàn"
}
}).attach()
}
}<file_sep>package com.example.tttkotlin.model
data class User (val user: String, val pass: String)<file_sep>package com.example.tttkotlin.util
class Constants {
companion object{
const val BASE_URL = "https://apithaytru.herokuapp.com/"
}
}<file_sep>package com.example.tttkotlin.activity
import android.os.AsyncTask
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.util.Log
import android.view.View
import android.widget.RatingBar
import android.widget.Toast
import coil.api.load
import com.example.tttkotlin.R
import com.example.tttkotlin.api.RetrofitInstance
import com.example.tttkotlin.model.MauTin
import com.example.tttkotlin.model.Status
import com.facebook.shimmer.ShimmerFrameLayout
import kotlinx.android.synthetic.main.activity_body.*
import org.json.JSONArray
import org.json.JSONObject
import retrofit2.Call
import retrofit2.Callback
import retrofit2.Response
import java.io.BufferedReader
import java.io.InputStreamReader
import java.lang.Exception
import java.net.HttpURLConnection
import java.net.URL
class BodyActivity : AppCompatActivity() {
lateinit var mauTin: MauTin
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_body)
var actionBar = getSupportActionBar()
if (actionBar != null) {
actionBar.setDisplayHomeAsUpEnabled(true)
}
val id_post : String? = intent.getStringExtra("id_post")
//val a: String = "https://apisimpleappp.herokuapp.com/post/" + id_post
//ReadJSON().execute(url)
id_post?.let{
loadBodyMauTin(it)
}
// rtbDGBody.setOnRatingBarChangeListener(object : RatingBar.OnRatingBarChangeListener {
// override fun onRatingChanged(p0: RatingBar?, p1: Float, p2: Boolean) {
//// RetrofitInstance.instance.danhGiaBaiViet(rtbDGBody.getRating()).enqueue(object : Callback<ArrayList<MauTin>>{
//// override fun onResponse(call: Call<ArrayList<MauTin>>, response: Response<ArrayList<MauTin>>) {
// Toast.makeText(applicationContext,"Đánh giá "+ rtbDGBody.getRating().toString()+" sao thành công!", Toast.LENGTH_SHORT).show()
//// }
////
//// override fun onFailure(call: Call<ArrayList<MauTin>>, t: Throwable) {
//// Toast.makeText(applicationContext,"Đánh giá thất bại: "+ t, Toast.LENGTH_SHORT).show()
//// }
////
//// })
//
// }
// })
}
// fun tangViewNew(id_post: String){
// RetrofitInstance.instance.addView(id_post).enqueue(object : Callback<Status>{
// override fun onResponse(call: Call<Status>, response: Response<Status>) {
// response.body()?.let{
// //Toast.makeText(applicationContext,it.getStatus().toString(), Toast.LENGTH_SHORT).show()
// }
// }
// override fun onFailure(call: Call<Status>, t: Throwable) {
// //Toast.makeText(applicationContext,t.message, Toast.LENGTH_SHORT).show()
// }
// })
// }
fun loadBodyMauTin(id_post : String){
RetrofitInstance.instance.getMauTin(id_post).enqueue(object : Callback<ArrayList<MauTin>>{
override fun onResponse(call: Call<ArrayList<MauTin>>, response: Response<ArrayList<MauTin>>) {
response.body()?.let {
tvTieuDeBody.setText(it.get(0).getTieuDe())
tvNoiDungBody.setText(it.get(0).getNoiDung())
tvNgayTaoBody.setText((it.get(0).getSoPhut()).substring(0, 16))
imgHinhAnhBody.load(it.get(0).getHinhAnh())
tvView.setText(it.get(0).getDanhGia().toInt().toString()+" lượt xem")
tvTacGiaBody.setText(it.get(0).getNguoiTao())
//shimmerFrame.apply {
// stopShimmer()
// visibility = View.GONE
// }
}
}
override fun onFailure(call: Call<ArrayList<MauTin>>, t: Throwable) {
}
})
}
// override fun onResume() {
// super.onResume()
// shimmerFrame.startShimmer()
// }
// inner class ReadJSON : AsyncTask<String, Void, String>() {
// override fun doInBackground(vararg params: String?): String {
// var content: StringBuffer = StringBuffer()
// val url: URL = URL(params[0])
// val urlConnection: HttpURLConnection = url.openConnection() as HttpURLConnection
// var inputStreamReader: InputStreamReader = InputStreamReader(urlConnection.inputStream)
// val bufferedReader: BufferedReader = BufferedReader(inputStreamReader)
// var line: String = ""
// try {
// do {
// line = bufferedReader.readLine()
// if (line != null) {
// content.append(line)
// }
// } while (line != null)
// bufferedReader.close()
// } catch (e: Exception) {
// Log.d("loi", e.toString())
// }
// return content.toString()
// }
//
// override fun onPostExecute(result: String?) {
// super.onPostExecute(result)
// val jsonArray: JSONArray = JSONArray(result)
// val listMauTin: ArrayList<MauTin> = ArrayList()
// for (i in 0..jsonArray.length() - 1) {
// var jsonOb: JSONObject = jsonArray.getJSONObject(i)
// tvTieuDeBody.setText(jsonOb.getString("title"))
// tvNoiDungBody.setText(jsonOb.getString("content"))
// tvNgayTaoBody.setText((jsonOb.getString("create_time")).substring(0, 16))
// imgHinhAnhBody.load(jsonOb.getString("img"))
// rtbDGBody.setRating(jsonOb.getString("rating").toFloat());
// tvTacGiaBody.setText(jsonOb.getString("username"))
// }
// }
// }
}<file_sep>package com.example.tttkotlin
import android.content.Intent
import android.os.Bundle
import android.view.View
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
import com.example.tttkotlin.activity.BodyActivity
import com.example.tttkotlin.adapter.MauTinAdapter
import com.example.tttkotlin.adapter.MauTinAdapterSearch
import com.example.tttkotlin.api.RetrofitInstance
import com.example.tttkotlin.model.MauTin
import kotlinx.android.synthetic.main.activity_main.*
import kotlinx.android.synthetic.main.activity_search.*
import kotlinx.android.synthetic.main.fragment_bong_chuyen.view.*
import retrofit2.Call
import retrofit2.Callback
import retrofit2.Response
import java.util.*
class SearchActivity : AppCompatActivity() {
lateinit var listMauTin:ArrayList<MauTin>
lateinit var displayListMauTin:ArrayList<MauTin>
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_search)
listMauTin = arrayListOf()
displayListMauTin = arrayListOf()
val textSearch : String? = intent.getStringExtra("textSearch")
loadListView(textSearch.toString())
}
override fun onResume() {
super.onResume()
shimmerFrameSearch.startShimmer()
}
fun loadListView( test:String){
RetrofitInstance.instance.getListMauTin2().enqueue(object : Callback<ArrayList<MauTin>> {
override fun onResponse(
call: Call<ArrayList<MauTin>>,
response: Response<ArrayList<MauTin>>
) {
response.body()?.let { bao ->
listMauTin.addAll(bao)
displayListMauTin.clear()
listMauTin.forEach{
if(it.getTieuDe().contains(test, ignoreCase = true)){
displayListMauTin.add(it)
}
}
if(displayListMauTin.size>0){
val adapterMauTin = MauTinAdapterSearch(applicationContext, displayListMauTin)
listViewSearch.apply {
adapter = adapterMauTin
setOnItemClickListener { parent, view, position, id ->
showChiTietPost(
displayListMauTin.get(position).getIdMauTin().toString()
)
}
}
shimmerFrameSearch.apply {
stopShimmer()
visibility = View.GONE
}
}
else{
Toast.makeText(applicationContext, "Không có kết quả tìm kiếm!", Toast.LENGTH_SHORT).show()
}
}
}
override fun onFailure(call: Call<ArrayList<MauTin>>, t: Throwable) {
}
})
}
private fun showChiTietPost(id: String?) {
val intent = Intent(applicationContext, BodyActivity::class.java)
intent.putExtra("id_post", id)
startActivity(intent)
}
}<file_sep>package com.example.tttkotlin.adapter
import android.content.Context
import androidx.fragment.app.*
import androidx.viewpager2.adapter.FragmentStateAdapter
import com.example.tttkotlin.fragment.BoiLoiFragment
import com.example.tttkotlin.fragment.BongChuyenFragment
import com.example.tttkotlin.fragment.BongDaFragment
@Suppress("DEPRECATION")
class TabAdapter(fm: FragmentActivity, val list:MutableList<Fragment>): FragmentStateAdapter(fm) {
override fun getItemCount(): Int {
return list.size
}
override fun createFragment(position: Int): Fragment {
return list[position]
}
}<file_sep>package com.example.tttkotlin.model
import com.google.gson.annotations.SerializedName
class Status {
@SerializedName("status")
private var status:Int = 0
constructor(st: Int) {
this.status = st
}
fun getStatus(): Int{
return status
}
}<file_sep>package com.example.tttkotlin.model
import com.google.gson.annotations.SerializedName
public class MauTin {
@SerializedName("id_post")
private var idMauTin=0;
@SerializedName("img")
private var hinhAnh="";
@SerializedName("title")
private var tieuDe="";
@SerializedName("create_time")
private var soPhut="";
@SerializedName("content")
private var noidung="";
@SerializedName("rating")
private var danhgia =0.0;
@SerializedName("username")
private var nguoiTao="";
constructor(idMauTin: Int, hinhAnh: String, tieuDe: String, soPhut: String) {
this.idMauTin = idMauTin
this.hinhAnh = hinhAnh
this.tieuDe = tieuDe
this.soPhut = soPhut
}
constructor(idMauTin: Int, hinhAnh: String, tieuDe: String, soPhut: String, noiDung:String, danhGia:Float, nguoiTao : String) {
this.idMauTin = idMauTin
this.hinhAnh = hinhAnh
this.tieuDe = tieuDe
this.soPhut = soPhut
this.noidung = noiDung
this.danhgia = danhGia.toDouble()
this.nguoiTao = nguoiTao
}
fun setidMauTin(id: Int){
idMauTin = id
}
fun setHinhAnh(ha: String){
hinhAnh = ha
}
fun setTieuDe(td: String){
tieuDe = td
}
fun setSoPhut(sp: String){
soPhut = sp
}
fun getIdMauTin(): Int{
return idMauTin
}
fun getHinhAnh(): String{
return hinhAnh
}
fun getTieuDe(): String{
return tieuDe
}
fun getNoiDung(): String{
return noidung
}
fun getDanhGia(): Double{
return danhgia
}
fun getNguoiTao(): String{
return nguoiTao
}
fun getSoPhut(): String{
return soPhut
}
}<file_sep>package com.example.tttkotlin.activity
import android.app.ActivityOptions
import android.content.Context
import android.content.Intent
import android.content.SharedPreferences
import android.database.sqlite.SQLiteDatabase.create
import android.os.Build
import android.os.Bundle
import android.text.method.HideReturnsTransformationMethod
import android.text.method.PasswordTransformationMethod
import android.view.View
import android.view.animation.AnimationUtils
import java.util.Objects;
import android.widget.Toast
import androidx.annotation.RequiresApi
import androidx.appcompat.app.AppCompatActivity
import androidx.core.util.Pair.create
import com.example.tttkotlin.R
import com.example.tttkotlin.api.RetrofitInstance
import com.example.tttkotlin.model.Login
import kotlinx.android.synthetic.main.activity_dang_ky_t_k.*
import kotlinx.android.synthetic.main.activity_login.*
import kotlinx.android.synthetic.main.activity_login.checkShowPass
import kotlinx.android.synthetic.main.activity_login.cvDNLogin
import kotlinx.android.synthetic.main.activity_login.edtEmailLogin
import kotlinx.android.synthetic.main.activity_login.edtPassLogin
import kotlinx.android.synthetic.main.activity_login_new.*
import kotlinx.android.synthetic.main.nav_header.*
import retrofit2.Call
import retrofit2.Callback
import retrofit2.Response
import java.net.URI.create
class LoginActivity : AppCompatActivity() {
@RequiresApi(Build.VERSION_CODES.LOLLIPOP)
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_login_new)
val animation = AnimationUtils.loadAnimation(this,R.anim.uptodowndiagonal)
rLayoutDN.setAnimation(animation)
val animationx = AnimationUtils.loadAnimation(this,R.anim.tranlation_x)
edtEmailLogin.setAnimation(animationx)
tvEmailDN.setAnimation(animationx)
edtPassLogin.setAnimation(animationx)
tvPassDN.setAnimation(animationx)
checkShowPass.setAnimation(animationx)
val animationy = AnimationUtils.loadAnimation(this,R.anim.tranlation_y)
rLayoutAdd.setAnimation(animationy)
checkShowPass.setOnClickListener{
if(checkShowPass.isChecked) {
edtPassLogin.transformationMethod = HideReturnsTransformationMethod.getInstance()
} else {
edtPassLogin.transformationMethod = PasswordTransformationMethod.getInstance()
}
}
cvDNLogin.setOnClickListener {
val email = edtEmailLogin.text.toString().trim()
val pass = edtPassLogin.text.toString().trim()
if(email.isEmpty()){
edtEmailLogin.error = "Vui lòng kiểm tra lại tài khoản!"
edtEmailLogin.requestFocus()
return@setOnClickListener
}
if(pass.isEmpty()){
edtPassLogin.error = "Vui lòng kiểm tra lại mật khẩu!"
edtPassLogin.requestFocus()
return@setOnClickListener
}
RetrofitInstance.instance.userLogin(email, pass).enqueue(object : Callback<Login> {
override fun onResponse(call: Call<Login>, response: Response<Login>) {
val sharedPreferences: SharedPreferences = getSharedPreferences(
"dataLogin",
Context.MODE_PRIVATE
)
var editor = sharedPreferences.edit()
if (!response.body()?.getAccessToken().equals(null)) {
editor.apply {
putString("email", email)
putString("token", response.body()?.getAccessToken())
putString("pass", <PASSWORD>)
putString("username", response.body()?.getUserName())
}.apply()
editor.commit()
Toast.makeText(
applicationContext,
"Đăng nhập thành công!",
Toast.LENGTH_SHORT
).show()
val intent = Intent(applicationContext, MainActivity::class.java)
startActivity(intent)
onBackPressed()
} else {
Toast.makeText(
applicationContext,
"Đăng nhập thất bại!",
Toast.LENGTH_SHORT
).show()
}
}
override fun onFailure(call: Call<Login>, t: Throwable) {
Toast.makeText(
applicationContext,
"Vui lòng kiểm tra lại tài khoản hoặc mật khẩu!",
Toast.LENGTH_SHORT
).show()
}
})
}
imgbtnDKTK.setOnClickListener {
val intent = Intent(applicationContext, DangKyTKActivity::class.java)
startActivity(intent)
}
// tvDKTKNew.setOnClickListener {
// val intent = Intent(applicationContext, DangKyTKActivity::class.java)
// startActivity(intent)
// }
}
}<file_sep>package com.example.tttkotlin.model
import com.google.gson.annotations.SerializedName
class DanhMuc {
@SerializedName("id_category")
private var id_category = 0
@SerializedName("name")
private var name="";
@SerializedName("id_parent")
private var id_parent="";
@SerializedName("level")
private var level=0;
constructor(id_category: Int, name: String, id_parent: String, level: Int) {
this.id_category = id_category
this.name = name
this.id_parent = id_parent
this.level = level
}
fun setidDanhMuc(id: Int){
id_category = id
}
fun setName(ha: String){
name = ha
}
fun setidParent(td: String){
id_parent = td
}
fun setLevel(sp: Int){
level = sp
}
fun getIdDanhMuc(): Int{
return id_category
}
fun getName(): String{
return name
}
fun getIdParent(): String{
return id_parent
}
fun getLevel(): Int{
return level
}
}<file_sep>package com.example.tttkotlin.api
import com.example.tttkotlin.model.DanhMuc
import com.example.tttkotlin.model.Login
import com.example.tttkotlin.model.MauTin
import com.example.tttkotlin.model.Status
import retrofit2.Call
import retrofit2.http.*
interface SimpleApi {
@GET("post?state=id_category=1,status=1&fields=id_post,title,img,create_time&limit=10&sort=create_time,desc")
fun getListMauTin1() : Call<ArrayList<MauTin>>
@GET("post?state=id_category=2,status=1&fields=id_post,title,img,create_time&limit=10&sort=create_time,desc")
fun getListMauTin2() : Call<ArrayList<MauTin>>
@GET("post?state=id_category=3,status=1&fields=id_post,title,img,create_time&limit=10&sort=create_time,desc")
fun getListMauTin3() : Call<ArrayList<MauTin>>
@GET("category")
fun getListNameDanhMuc() : Call<ArrayList<DanhMuc>>
@GET("post/{id}")
fun getMauTin(@Path("id") id :String) : Call<ArrayList<MauTin>>
@GET("login")
fun userLogin(@Header ("email") user : String, @Header("password") pass : String) : Call<Login>
@POST("post/view/{id}")
fun addView(@Path("id") id :String) : Call<Status>
@POST("account/reg")
fun dangKyTaiKhoan(@Header ("username") username : String, @Header ("email") email : String, @Header("password") pass : String) : Call<Status>
}<file_sep>package com.example.tttkotlin.model
import com.google.gson.annotations.SerializedName
class Login {
@SerializedName("access_token")
private var access_token="";
@SerializedName("refresh_token")
private var refresh_token="";
@SerializedName("email")
private var email="";
@SerializedName("username")
private var username="";
fun getAccessToken(): String{
return access_token
}
fun getRefreshToken(): String{
return refresh_token
}
fun getUserName(): String{
return username
}
fun getEmail(): String{
return email
}
}<file_sep>package com.example.tttkotlin.api
import retrofit2.Retrofit
import retrofit2.converter.gson.GsonConverterFactory
import com.example.tttkotlin.util.Constants.Companion.BASE_URL
object RetrofitInstance {
val instance : SimpleApi by lazy {
val retrofit = Retrofit.Builder()
.baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.build()
retrofit.create(SimpleApi::class.java)
}
}<file_sep>package com.example.tttkotlin.adapter
import android.content.Context
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.BaseAdapter
import android.widget.ImageView
import android.widget.TextView
import coil.api.load
import coil.size.Scale
import com.example.tttkotlin.R
import com.example.tttkotlin.model.MauTin
import java.text.DateFormat
import java.text.SimpleDateFormat
import java.util.*
import kotlin.collections.ArrayList
class MauTinAdapterSearch(var context : Context, var listMauTin:ArrayList<MauTin>): BaseAdapter() {
override fun getCount(): Int {
return listMauTin.size
}
override fun getItem(position: Int): Any {
return listMauTin.get(position)
}
override fun getItemId(position: Int): Long {
return 0
}
override fun getView(position: Int, convertView: View?, parent: ViewGroup?): View {
var viewMauTin: View?
var layoutInflater : LayoutInflater = LayoutInflater.from(context)
viewMauTin = layoutInflater.inflate(R.layout.item_list_view, null)
val mautin : MauTin = getItem(position) as MauTin
var imgV : ImageView = viewMauTin.findViewById(R.id.img)
imgV.load(mautin.getHinhAnh()){
placeholder(android.R.drawable.ic_menu_gallery)
}
var tvT : TextView = viewMauTin.findViewById(R.id.txtTittle)
tvT.setText(mautin.getTieuDe())
var tgianTao : String = mautin.getSoPhut().substring(0,18)
var df : DateFormat = SimpleDateFormat("yyyy-MM-dd HH:mm:ss")
var tgianHT :String = df.format(Calendar.getInstance().getTime())
var format : SimpleDateFormat = SimpleDateFormat("yyyy-MM-dd HH:mm:ss")
var date1 = format.parse(tgianTao)
var date2 = format.parse(tgianHT)
var diff : Long = 0
diff = date2.getTime() - date1.getTime()
var diffSeconds :Long = diff / 1000
var diffMinutes :Long = diff / (60 * 1000)
var diffHours :Long = diff / (60 * 60 * 1000)
var ngay :Long = diff / (60 * 60 * 1000*24)
var thang :Long = diff / (60 * 60 * 1000*24*30)
if(thang<0){
thang=thang*(-1)
}
var nam :Long = diff / (60 * 60 * 1000*24*30*12)
if(nam<0){
nam=nam*(-1)
}
var tvTime : TextView = viewMauTin.findViewById(R.id.txtTime)
if(diffSeconds<60){
tvTime.setText(diffSeconds.toString() + " giây trước")
}
if(diffSeconds>60&&diffMinutes<60){
tvTime.setText(diffMinutes.toString() +" phút trước")
}
if(diffMinutes>60 && diffHours<24){
tvTime.setText(diffHours.toString() +" giờ trước")
}
if(diffHours>24 && ngay<30){
tvTime.setText(ngay.toString() +" ngày trước")
}
if(ngay>30 && thang<12){
tvTime.setText(thang.toString() +" tháng trước")
}
if(thang>12&&nam<100){
tvTime.setText(nam.toString() +" năm trước")
}
return viewMauTin
}
}<file_sep>package com.example.tttkotlin.fragment
import android.content.Intent
import android.os.Bundle
import android.view.Display
import androidx.fragment.app.Fragment
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.ListView
import android.widget.SearchView
import android.widget.Toast
import com.example.tttkotlin.R
import com.example.tttkotlin.activity.BodyActivity
import com.example.tttkotlin.model.MauTin
import com.example.tttkotlin.adapter.MauTinAdapter
import com.example.tttkotlin.api.RetrofitInstance
import kotlinx.android.synthetic.main.activity_login_new.view.*
import kotlinx.android.synthetic.main.activity_main.*
import kotlinx.android.synthetic.main.fragment_bong_chuyen.view.*
import retrofit2.Call
import retrofit2.Callback
import retrofit2.Response
import java.util.*
import kotlin.collections.ArrayList
class BongChuyenFragment : Fragment() {
lateinit var listMauTin:ArrayList<MauTin>
lateinit var viewBongChuyen:View
override fun onCreateView(
inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?): View {
viewBongChuyen = inflater.inflate(R.layout.fragment_bong_chuyen, container, false)
listMauTin = arrayListOf()
loadListView()
return viewBongChuyen
}
override fun onResume() {
super.onResume()
viewBongChuyen.shimmerFrame.startShimmer()
}
fun loadListView(){
RetrofitInstance.instance.getListMauTin2().enqueue(object:Callback<ArrayList<MauTin>>{
override fun onResponse(call: Call<ArrayList<MauTin>>, response: Response<ArrayList<MauTin>>) {
response.body()?.let { bao->
listMauTin.addAll(bao)
val adapterMauTin = MauTinAdapter(requireContext(), listMauTin)
viewBongChuyen.apply {
listViewMauTinBC.apply {
adapter = adapterMauTin
setOnItemClickListener { parent, view, position, id ->
showChiTietPost(listMauTin.get(position).getIdMauTin().toString())
}
}
shimmerFrame.apply {
stopShimmer()
visibility = View.GONE
}
}
}
}
override fun onFailure(call: Call<ArrayList<MauTin>>, t: Throwable) {
}
})
}
private fun showChiTietPost(id: String?) {
val intent = Intent(context, BodyActivity::class.java)
intent.putExtra("id_post", id)
requireActivity().startActivity(intent)
}
}<file_sep>package com.example.tttkotlin.fragment
import android.content.Intent
import android.os.Bundle
import androidx.fragment.app.Fragment
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.ListView
import com.example.tttkotlin.R
import com.example.tttkotlin.activity.BodyActivity
import com.example.tttkotlin.adapter.MauTinAdapter
import com.example.tttkotlin.model.MauTin
import com.example.tttkotlin.api.RetrofitInstance
import kotlinx.android.synthetic.main.fragment_bong_da.*
import kotlinx.android.synthetic.main.fragment_bong_da.view.*
import retrofit2.Call
import retrofit2.Callback
import retrofit2.Response
class BongDaFragment : Fragment() {
lateinit var listMauTin:ArrayList<MauTin>
lateinit var listView:ListView
lateinit var viewBongDaFragment:View
override fun onCreateView(
inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
// Inflate the layout for this fragment
// val url : String = "https://apisimpleappp.herokuapp.com/post?state=id_category=2,status=1&fields=id_post,title,img,create_time&limit=14&sort=create_time,desc"
// ReadJSON().execute(url)
viewBongDaFragment = inflater.inflate(R.layout.fragment_bong_da, container, false)
listMauTin = arrayListOf()
loadListView()
return viewBongDaFragment
}
fun loadListView(){
RetrofitInstance.instance.getListMauTin1().enqueue(object : Callback<ArrayList<MauTin>>{
override fun onResponse(call: Call<ArrayList<MauTin>>, response: Response<ArrayList<MauTin>>) {
response.body()?.let { list ->
listMauTin.addAll(list)
val adapterMauTin = MauTinAdapter(requireContext(), listMauTin)
viewBongDaFragment.apply {
listViewMauTinBD.apply {
adapter = adapterMauTin
setOnItemClickListener { parent, view, position, id ->
showChiTietPost(listMauTin.get(position).getIdMauTin().toString())
}
}
shimmerFrame.apply {
stopShimmer()
visibility = View.GONE
}
}
}
}
override fun onFailure(call: Call<ArrayList<MauTin>>, t: Throwable) {
}
})
}
override fun onResume() {
super.onResume()
viewBongDaFragment.shimmerFrame.startShimmer()
}
// inner class ReadJSON : AsyncTask<String, Void, String>() {
// override fun doInBackground(vararg params: String?): String {
// var content: StringBuffer = StringBuffer()
// val url: URL = URL(params[0])
// val urlConnection: HttpURLConnection = url.openConnection() as HttpURLConnection
// val inputStreamReader = InputStreamReader(urlConnection.inputStream)
// val bufferedReader = BufferedReader(inputStreamReader)
// var line: String = ""
// try {
// do {
// line = bufferedReader.readLine()
// if (line != null) {
// content.append(line)
// }
// } while (line != null)
// bufferedReader.close()
// } catch (e: Exception) {
// Log.d("loi", e.toString())
// }
// return content.toString()
// }
//
// override fun onPostExecute(result: String?) {
// super.onPostExecute(result)
// val jsonArray: JSONArray = JSONArray(result)
// val listMauTin: ArrayList<MauTin> = ArrayList()
// for (i in 0..jsonArray.length() - 1) {
// var jsonOb: JSONObject = jsonArray.getJSONObject(i)
// var id = jsonOb.getInt("id_post")
// var tieuDe = jsonOb.getString("title")
//
// var img = jsonOb.getString("img")
// var soPhut = jsonOb.getString("create_time")
// var mauTin: MauTin = MauTin(id, img, tieuDe, soPhut)
// listMauTin.add(mauTin)
// }
// var adapterMauTin = MauTinAdapter(context!!, listMauTin)
// listview.adapter = adapterMauTin
// listview.setOnItemClickListener { parent, view, position, id ->
// showChiTietPost(listMauTin.get(position).getIdMauTin().toString())
// }
// }
//
// }
private fun showChiTietPost(id: String?) {
val intent = Intent(context, BodyActivity::class.java)
intent.putExtra("id_post", id)
requireActivity().startActivity(intent)
}
} | ed5194601377f76ae016aa5df8da993839e53943 | [
"Kotlin"
] | 18 | Kotlin | nhatquan1702/TinTheThao | f2e45977e75f140658d36d6579506edeb750da38 | ebb752aa3275be8147ec260540df8e46ee1c363d |
refs/heads/master | <repo_name>Taughran23/communiteerFrontEnd<file_sep>/src/components/MyGroups.js
import React from 'react';
import { StyleSheet, Text, View, Image, Linking } from 'react-native';
import { Actions } from 'react-native-router-flux';
const MyGroups = () => {
return (
<View style={styles.container}>
<Text
style={styles.groups}>
MY GROUPS
</Text>
</View>
)
}
const styles = {
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#bb0000'
},
groups: {
fontSize: 20,
textAlign: 'center',
margin: 10,
color: '#ffffff'
}
}
export default MyGroups<file_sep>/src/components/ProfilePage.js
import React, { Component } from 'react';
import { connect } from 'react-redux'
import { Text, View, Image } from 'react-native';
import { FetchUserData } from '../actions'
import { Card, CardSection } from './common';
import { Actions } from 'react-native-router-flux';
import * as actions from '../actions';
// hard coded user id - get this at log in???
const userId = 1;
class ProfilePage extends Component {
componentDidMount() {
this.props.fetchUserData()
}
render() {
return (
<Card style={styles.containerStyle}>
<CardSection>
<Image
style={styles.imageStyle}
source={{uri: this.props.user.profilePicture}} />
</CardSection>
<CardSection>
<View style={styles.headerContentStyle}>
<Text>Hello {this.props.user.user_fName}</Text>
</View>
</CardSection>
<Text onPress={() => Actions.addGroup()}>Add Group</Text>
<Text onPress={() => Actions.addEvent()}>Add Event</Text>
</Card>
)
}
};
const mapDispatchToProps = (dispatch) => {
return {
fetchUserData: () => {
dispatch(actions.fetchUserData(userId))
}
}
}
const mapStateToProps = (state) => {
return {
user: state.user
}
}
const styles = {
containerStyle: {
paddingTop: 30,
backgroundColor: '#bb0000'
},
headerContentStyle: {
flexDirection: 'column',
justifyContent: 'space-around'
},
headerTextStyle: {
fontSize: 18
},
thumbnailStyle: {
height: 50,
width: 50
},
thumbnailContainerStyle: {
justifyContent: 'center',
alignItems: 'center',
marginLeft: 10,
marginRight: 10
},
imageStyle: {
height: 300,
flex: 1,
width: null
}
}
export default connect(mapStateToProps, mapDispatchToProps)(ProfilePage); | 36aa976fb63389478e7d3d29fc896c1c758aff59 | [
"JavaScript"
] | 2 | JavaScript | Taughran23/communiteerFrontEnd | 48ffd3125b160339dc960040619a97849ccc6844 | b875f45721d9a4a98264bd0f19ef1dcb1cc93d28 |
refs/heads/master | <file_sep>import React, { Component } from 'react'
import { Platform, Text, Linking } from 'react-native';
import { NavigationActions } from 'react-navigation';
class Profile extends Component{
static navigationOptions = {
title: 'Profile',
};
componentDidMount() {
if (Platform.OS === 'android') {
Linking.getInitialURL().then(url => {
this.navigate(url);
});
} else {
Linking.addEventListener('url', this.handleOpenURL);
}
}
componentWillUnmount() {
Linking.removeEventListener('url', this.handleOpenURL);
}
handleOpenURL = (event) => {
this.navigate(event.url);
}
navigate = (url) => {
const route = url.replace(/.*?:\/\//g, '');
const routeName = route.split('/')[2];
if (routeName === 'details') {
this.props.navigation.navigate('ProfileNav',{},NavigationActions.navigate({ routeName: 'Deatils' }))
} else if (routeName === 'photos') {
this.props.navigation.navigate('ProfileNav',{},NavigationActions.navigate({ routeName: 'Photos' }))
};
}
render(){
return(
<Text>Profile</Text>
)
}
}
export default Profile;<file_sep>import React, { Component } from 'react'
import { Text } from 'react-native';
import { createAppContainer, createStackNavigator, createBottomTabNavigator } from 'react-navigation';
import Deatils from './details';
import Profile from './profile';
import Photos from './photos';
const ProfileNav = createBottomTabNavigator({
Profile: { screen: Profile },
Deatils: { screen: Deatils },
Photos: { screen: Photos}
},{
initialRouteName : 'Profile',
//headerMode : 'none'
});
export default createAppContainer(ProfileNav);<file_sep>/**
* Sample React Native App
* https://github.com/facebook/react-native
*
* @format
* @flow
*/
import React, {Component} from 'react';
import {Platform, StyleSheet, Text, View} from 'react-native';
const instructions = Platform.select({
ios: 'Press Cmd+R to reload,\n' + 'Cmd+D or shake for dev menu',
android:
'Double tap R on your keyboard to reload,\n' +
'Shake or press menu button for dev menu',
});
import { createAppContainer, createStackNavigator, createBottomTabNavigator } from 'react-navigation';
import Home from './src/home';
import ProfileNavContainer from './src/profileNav';
import Setting from './src/setting';
import Deatils from './src/details';
import Profile from './src/profile';
import Photos from './src/photos';
const App = createStackNavigator({
Home: { screen: Home },
ProfileNav: { screen: createBottomTabNavigator({
Profile: { screen: Profile },
Deatils: { screen: Deatils },
Photos: { screen: Photos}
},{
initialRouteName : 'Profile',
//headerMode : 'none'
}) },
Setting: { screen: Setting },
},{
initialRouteName : 'Home'
});
export default createAppContainer(App);<file_sep># deeplinking
# deeplinking
<file_sep>import React, { Component } from 'react'
import { Text } from 'react-native';
class Photos extends Component{
static navigationOptions = {
title: 'Photos',
};
render(){
return(
<Text>Photos</Text>
)
}
}
export default Photos;<file_sep>import React, { Component } from 'react'
import { Text, Platform, Linking } from 'react-native';
class Home extends Component{
static navigationOptions = {
title: 'Home',
};
componentDidMount() {
if (Platform.OS === 'android') {
Linking.getInitialURL().then(url => {
this.navigate(url);
});
} else {
Linking.addEventListener('url', this.handleOpenURL);
}
}
componentWillUnmount() {
Linking.removeEventListener('url', this.handleOpenURL);
}
handleOpenURL = (event) => {
this.navigate(event.url);
}
navigate = (url) => {
const { navigate } = this.props.navigation;
const route = url.replace(/.*?:\/\//g, '');
const routeName = route.split('/')[1];
//alert(routeName);
if (routeName === 'profile') {
navigate('ProfileNav',{} )
} else if (routeName === 'setting') {
navigate('Setting',{} )
} ;
}
render(){
return(
<Text>Home</Text>
)
}
}
export default Home; | 60e2224a22a0741a59bbf7de692c61a3e4f68b1b | [
"JavaScript",
"Markdown"
] | 6 | JavaScript | arpitsharma11/deeplinking | c555e47c4080e69799787cd1d6c736e6da93eebc | 578f6cc843d25eb54cb741d3e812fa891d4d6d99 |
refs/heads/main | <file_sep># awesome_rush_b_webapp
## DevLog
### 1/22/2021 - Zixiao
1. package related modification
* Add ```config```, ```util```
* ```config``` is used for ```@Configuration``` class
* ```util``` is used for common tool class like jwt.
2. test related modification
* Add ```SubscriberControllerTest```
3. dependency related modeification
* Add ```junit``` dependency
4. **BUG**
* ```mapper``` All insert methods need to be change. **Because the value of PK cannot be retrieved**
Take ```UserMapper``` as an example:
```java
// Bug code
@Insert("insert into user(userId, username, password, email) values " +
"(UUID(), #{username}, #{password}, #{email})")
void save(User user);
// Correct code: user @SelectKey to retrieve the PK
// Reference:
// https://mybatis.org/mybatis-3/apidocs/org/apache/ibatis/annotations/SelectKey.html
// https://www.cnblogs.com/loveLands/articles/10099768.html
@SelectKey(statement = "SELECT UUID()", keyProperty = "userId", before = true, resultType = String.class)
@Insert("insert into user(userId, username, password, email) values " +
"(#{userId}, #{username}, #{password}, #{email})")
void save(User user);
```
### 1/26/2021 - jonah
1. Implemented test classes for DAO classes, and user service
2. Minor change has been made for Dao impl and tag Mapper.
3. All test succeed for DAO classes and Service classes
### 2/1/2021 - jonah
1. added 404 handler in controller for the resource not found<file_sep>package rushb.webapp.model;
import lombok.Data;
@Data
public class Tag {
private String tagId;
private String name;
private int count;
}
| cd80dc10c16668bf25ef4f1a73b2134d841c4e33 | [
"Markdown",
"Java"
] | 2 | Markdown | JonahLuo/awesome_rush_b_webapp | 1303069f3eeb4f1ee8f1bf3751a30b23eaf67e58 | 38c98cc85f9809f9b4844c3cc732337a18b6deda |
refs/heads/master | <repo_name>inseev/etchingexpressions<file_sep>/assets/product-control.js
function loadProducts(){
return [
{
"product": {
"id": 2280280195,
"title": "Kickstarter - DM1 Engraving",
"body_html": "",
"vendor": "Decadent Minimalist",
"product_type": "",
"created_at": "2015-08-25T22:29:34-07:00",
"handle": "dm1-kickstarter-engraving",
"updated_at": "2015-08-29T10:00:01-07:00",
"published_at": "2015-08-28T11:30:00-07:00",
"template_suffix": "",
"published_scope": "web",
"tags": "",
"variants": [{
"id": 6542377603,
"product_id": 2280280195,
"title": "Custom - Included in reward",
"price": "0.00",
"sku": "",
"position": 1,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Custom - Included in reward",
"option2": null,
"option3": null,
"created_at": "2015-08-25T22:29:34-07:00",
"updated_at": "2015-09-01T20:00:52-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 995,
"old_inventory_quantity": 995,
"image_id": 4646402051,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6542377667,
"product_id": 2280280195,
"title": "No Agenda - Included in reward",
"price": "0.00",
"sku": "",
"position": 2,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "No Agenda - Included in reward",
"option2": null,
"option3": null,
"created_at": "2015-08-25T22:29:34-07:00",
"updated_at": "2015-08-28T11:02:40-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 999,
"old_inventory_quantity": 999,
"image_id": 4646403459,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6542377731,
"product_id": 2280280195,
"title": "Custom - Add On",
"price": "12.00",
"sku": "",
"position": 3,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Custom - Add On",
"option2": null,
"option3": null,
"created_at": "2015-08-25T22:29:34-07:00",
"updated_at": "2015-09-01T22:26:38-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 976,
"old_inventory_quantity": 976,
"image_id": 4646402051,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6542377795,
"product_id": 2280280195,
"title": "None",
"price": "0.00",
"sku": "",
"position": 4,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "None",
"option2": null,
"option3": null,
"created_at": "2015-08-25T22:29:34-07:00",
"updated_at": "2015-09-02T12:41:42-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 815,
"old_inventory_quantity": 815,
"image_id": 4646457155,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6633547075,
"product_id": 2280280195,
"title": "No Engraving - Carbon",
"price": "0.00",
"sku": "",
"position": 5,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "No Engraving - Carbon",
"option2": null,
"option3": null,
"created_at": "2015-08-27T13:51:02-07:00",
"updated_at": "2015-09-01T16:40:16-07:00",
"requires_shipping": true,
"taxable": false,
"barcode": "",
"inventory_quantity": 995,
"old_inventory_quantity": 995,
"image_id": null,
"weight": 0,
"weight_unit": "lb"
}],
"options": [{
"id": 2748414595,
"product_id": 2280280195,
"name": "Engraving",
"position": 1,
"values": [
"Custom - Included in reward",
"No Agenda - Included in reward",
"Custom - Add On",
"None",
"No Engraving - Carbon"
]
}],
"images": [{
"id": 4646402051,
"product_id": 2280280195,
"position": 1,
"created_at": "2015-08-25T22:29:35-07:00",
"updated_at": "2015-08-25T22:29:35-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/laser-engraving.jpg?v=1440566975",
"variant_ids": [
6542377603,
6542377731
]
}, {
"id": 4646403459,
"product_id": 2280280195,
"position": 2,
"created_at": "2015-08-25T22:29:39-07:00",
"updated_at": "2015-08-25T22:29:39-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/no-agenda.png?v=1440566979",
"variant_ids": [
6542377667
]
}, {
"id": 4646457155,
"product_id": 2280280195,
"position": 3,
"created_at": "2015-08-25T22:32:09-07:00",
"updated_at": "2015-08-25T22:32:09-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/none.png?v=1440567129",
"variant_ids": [
6542377795
]
}],
"image": {
"id": 4646402051,
"product_id": 2280280195,
"position": 1,
"created_at": "2015-08-25T22:29:35-07:00",
"updated_at": "2015-08-25T22:29:35-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/laser-engraving.jpg?v=1440566975",
"variant_ids": [
6542377603,
6542377731
]
}
}
}, {
"product": {
"id": 2280223619,
"title": "Kickstarter - RFID Blocking Card",
"body_html": "",
"vendor": "Decadent Minimalist",
"product_type": "",
"created_at": "2015-08-25T22:22:52-07:00",
"handle": "dm1-kickstarter-rfid-blocker",
"updated_at": "2015-08-29T10:02:50-07:00",
"published_at": "2015-08-28T11:31:00-07:00",
"template_suffix": "",
"published_scope": "web",
"tags": "",
"variants": [{
"id": 6541979011,
"product_id": 2280223619,
"title": "None",
"price": "0.00",
"sku": "",
"position": 1,
"grams": 0,
"inventory_policy": "deny",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "None",
"option2": null,
"option3": null,
"created_at": "2015-08-25T22:22:52-07:00",
"updated_at": "2015-09-02T12:41:42-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": -188,
"old_inventory_quantity": -188,
"image_id": 4646484419,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6541978947,
"product_id": 2280223619,
"title": "1 card",
"price": "10.00",
"sku": "",
"position": 2,
"grams": 0,
"inventory_policy": "deny",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "1 card",
"option2": null,
"option3": null,
"created_at": "2015-08-25T22:22:52-07:00",
"updated_at": "2015-08-30T20:55:34-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": -4,
"old_inventory_quantity": -4,
"image_id": 4646297411,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6544640067,
"product_id": 2280223619,
"title": "2 cards",
"price": "15.00",
"sku": "",
"position": 3,
"grams": 0,
"inventory_policy": "deny",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "2 cards",
"option2": null,
"option3": null,
"created_at": "2015-08-25T23:42:49-07:00",
"updated_at": "2015-09-01T19:39:30-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": -13,
"old_inventory_quantity": -13,
"image_id": 4646297411,
"weight": 0,
"weight_unit": "lb"
}],
"options": [{
"id": 2748356035,
"product_id": 2280223619,
"name": "RFID Option",
"position": 1,
"values": [
"None",
"1 card",
"2 cards"
]
}],
"images": [{
"id": 4646297411,
"product_id": 2280223619,
"position": 1,
"created_at": "2015-08-25T22:22:53-07:00",
"updated_at": "2015-08-25T22:22:53-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/DM1-RFID.jpg?v=1440566573",
"variant_ids": [
6541978947,
6544640067
]
}, {
"id": 4646484419,
"product_id": 2280223619,
"position": 2,
"created_at": "2015-08-25T22:33:09-07:00",
"updated_at": "2015-08-25T22:33:09-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/none_6125c6d3-bf4b-4646-a6c3-a04882c0e68a.png?v=1440567189",
"variant_ids": [
6541979011
]
}],
"image": {
"id": 4646297411,
"product_id": 2280223619,
"position": 1,
"created_at": "2015-08-25T22:22:53-07:00",
"updated_at": "2015-08-25T22:22:53-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/DM1-RFID.jpg?v=1440566573",
"variant_ids": [
6541978947,
6544640067
]
}
}
}, {
"product": {
"id": 2280353923,
"title": "Kickstarter - DMC Money Clip v2",
"body_html": "",
"vendor": "Decadent Minimalist",
"product_type": "",
"created_at": "2015-08-25T22:37:03-07:00",
"handle": "dm1-kickstarter-money-clip",
"updated_at": "2015-08-29T10:02:14-07:00",
"published_at": "2015-08-28T11:30:00-07:00",
"template_suffix": "",
"published_scope": "web",
"tags": "",
"variants": [{
"id": 6542749187,
"product_id": 2280353923,
"title": "None",
"price": "0.00",
"sku": "",
"position": 1,
"grams": 0,
"inventory_policy": "deny",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "None",
"option2": null,
"option3": null,
"created_at": "2015-08-25T22:37:03-07:00",
"updated_at": "2015-09-02T12:41:42-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": -176,
"old_inventory_quantity": -176,
"image_id": 4646561411,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6542749251,
"product_id": 2280353923,
"title": "Polished Stainless Steel",
"price": "25.00",
"sku": "",
"position": 2,
"grams": 0,
"inventory_policy": "deny",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Polished Stainless Steel",
"option2": null,
"option3": null,
"created_at": "2015-08-25T22:37:03-07:00",
"updated_at": "2015-09-02T08:21:06-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": -8,
"old_inventory_quantity": -8,
"image_id": 4646559747,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6542749379,
"product_id": 2280353923,
"title": "Titanium",
"price": "40.00",
"sku": "",
"position": 3,
"grams": 0,
"inventory_policy": "deny",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Titanium",
"option2": null,
"option3": null,
"created_at": "2015-08-25T22:37:03-07:00",
"updated_at": "2015-09-01T19:39:30-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": -23,
"old_inventory_quantity": -23,
"image_id": 4646561027,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6542749443,
"product_id": 2280353923,
"title": "Polished Stainless Steel - Included in Reward",
"price": "0.00",
"sku": "",
"position": 4,
"grams": 0,
"inventory_policy": "deny",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Polished Stainless Steel - Included in Reward",
"option2": null,
"option3": null,
"created_at": "2015-08-25T22:37:03-07:00",
"updated_at": "2015-08-29T15:44:22-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": -1,
"old_inventory_quantity": -1,
"image_id": 4646559747,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6542749507,
"product_id": 2280353923,
"title": "Titanium - Included in Reward",
"price": "0.00",
"sku": "",
"position": 5,
"grams": 0,
"inventory_policy": "deny",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Titanium - Included in Reward",
"option2": null,
"option3": null,
"created_at": "2015-08-25T22:37:03-07:00",
"updated_at": "2015-09-02T09:38:16-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 0,
"old_inventory_quantity": 0,
"image_id": 4646561027,
"weight": 0,
"weight_unit": "lb"
}],
"options": [{
"id": 2748489731,
"product_id": 2280353923,
"name": "Clip Design",
"position": 1,
"values": [
"None",
"Polished Stainless Steel",
"Titanium",
"Polished Stainless Steel - Backer Reward",
"Titanium - Backer Reward"
]
}],
"images": [{
"id": 4646559747,
"product_id": 2280353923,
"position": 1,
"created_at": "2015-08-25T22:37:04-07:00",
"updated_at": "2015-08-25T22:37:04-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/DMC-SS-Polish_3e988f62-ef31-4025-a1a3-b9f07fc188e7.jpeg?v=1440567424",
"variant_ids": [
6542749251,
6542749443
]
}, {
"id": 4646561027,
"product_id": 2280353923,
"position": 2,
"created_at": "2015-08-25T22:37:09-07:00",
"updated_at": "2015-08-25T22:37:09-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/DMC-Ti_7bcdadd5-fe1b-4e90-bf21-0e0b3bb1702a.jpeg?v=1440567429",
"variant_ids": [
6542749379,
6542749507
]
}, {
"id": 4646561411,
"product_id": 2280353923,
"position": 3,
"created_at": "2015-08-25T22:37:10-07:00",
"updated_at": "2015-08-25T22:37:10-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/none_b2ae2875-f82a-47b4-9e3c-92582a27c531.png?v=1440567430",
"variant_ids": [
6542749187
]
}],
"image": {
"id": 4646559747,
"product_id": 2280353923,
"position": 1,
"created_at": "2015-08-25T22:37:04-07:00",
"updated_at": "2015-08-25T22:37:04-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/DMC-SS-Polish_3e988f62-ef31-4025-a1a3-b9f07fc188e7.jpeg?v=1440567424",
"variant_ids": [
6542749251,
6542749443
]
}
}
}, {
"product": {
"id": 2279964611,
"title": "Kickstarter - Base DM1 Backer Reward - DO NOT REMOVE THIS ITEM",
"body_html": "This is only for people that backed the recent DM1 Kickstarter.",
"vendor": "Decadent Minimalist",
"product_type": "",
"created_at": "2015-08-25T22:02:16-07:00",
"handle": "base-dm1-kickstarter-backer-reward",
"updated_at": "2015-08-30T13:02:53-07:00",
"published_at": "2015-08-28T11:21:00-07:00",
"template_suffix": "",
"published_scope": "web",
"tags": "",
"variants": [{
"id": 6540945539,
"product_id": 2279964611,
"title": "Titanium / 4 Card / Brushed",
"price": "0.00",
"sku": "",
"position": 1,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Titanium",
"option2": "4 Card",
"option3": "Brushed",
"created_at": "2015-08-25T22:02:16-07:00",
"updated_at": "2015-09-01T17:22:04-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 987,
"old_inventory_quantity": 987,
"image_id": 4645789059,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6541044163,
"product_id": 2279964611,
"title": "Titanium / 4 Card / Stonewashed",
"price": "10.00",
"sku": "",
"position": 2,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Titanium",
"option2": "4 Card",
"option3": "Stonewashed",
"created_at": "2015-08-25T22:05:40-07:00",
"updated_at": "2015-08-31T07:52:11-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 998,
"old_inventory_quantity": 998,
"image_id": 4645789059,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6541017667,
"product_id": 2279964611,
"title": "Titanium / 4 Card / Ti Black",
"price": "20.00",
"sku": "",
"position": 3,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Titanium",
"option2": "4 Card",
"option3": "Ti Black",
"created_at": "2015-08-25T22:04:46-07:00",
"updated_at": "2015-09-02T01:45:32-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 993,
"old_inventory_quantity": 993,
"image_id": 4645789059,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6540945411,
"product_id": 2279964611,
"title": "Titanium / 8 Card / Brushed",
"price": "0.00",
"sku": "",
"position": 4,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Titanium",
"option2": "8 Card",
"option3": "Brushed",
"created_at": "2015-08-25T22:02:16-07:00",
"updated_at": "2015-09-02T12:40:31-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 910,
"old_inventory_quantity": 910,
"image_id": 4645789379,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6541044099,
"product_id": 2279964611,
"title": "Titanium / 8 Card / Stonewashed",
"price": "10.00",
"sku": "",
"position": 5,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Titanium",
"option2": "8 Card",
"option3": "Stonewashed",
"created_at": "2015-08-25T22:05:39-07:00",
"updated_at": "2015-09-01T17:48:45-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 995,
"old_inventory_quantity": 995,
"image_id": 4645789379,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6541017603,
"product_id": 2279964611,
"title": "Titanium / 8 Card / Ti Black",
"price": "20.00",
"sku": "",
"position": 6,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Titanium",
"option2": "8 Card",
"option3": "Ti Black",
"created_at": "2015-08-25T22:04:46-07:00",
"updated_at": "2015-09-01T20:00:52-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 981,
"old_inventory_quantity": 981,
"image_id": 4645789379,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6540945667,
"product_id": 2279964611,
"title": "Titanium / 12 Card / Brushed",
"price": "0.00",
"sku": "",
"position": 7,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Titanium",
"option2": "12 Card",
"option3": "Brushed",
"created_at": "2015-08-25T22:02:16-07:00",
"updated_at": "2015-09-02T12:41:42-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 951,
"old_inventory_quantity": 951,
"image_id": 4645789635,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6541044227,
"product_id": 2279964611,
"title": "Titanium / 12 Card / Stonewashed",
"price": "10.00",
"sku": "",
"position": 8,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Titanium",
"option2": "12 Card",
"option3": "Stonewashed",
"created_at": "2015-08-25T22:05:40-07:00",
"updated_at": "2015-08-30T13:29:28-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 995,
"old_inventory_quantity": 995,
"image_id": 4645789635,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6541017731,
"product_id": 2279964611,
"title": "Titanium / 12 Card / Ti Black",
"price": "20.00",
"sku": "",
"position": 9,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Titanium",
"option2": "12 Card",
"option3": "Ti Black",
"created_at": "2015-08-25T22:04:46-07:00",
"updated_at": "2015-09-01T20:00:52-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 989,
"old_inventory_quantity": 989,
"image_id": 4645789635,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6540945795,
"product_id": 2279964611,
"title": "Carbon Fiber / 4 Card / Gloss",
"price": "0.00",
"sku": "",
"position": 10,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Carbon Fiber",
"option2": "4 Card",
"option3": "Gloss",
"created_at": "2015-08-25T22:02:16-07:00",
"updated_at": "2015-08-29T15:34:16-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 999,
"old_inventory_quantity": 999,
"image_id": 4645789827,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6540945731,
"product_id": 2279964611,
"title": "Carbon Fiber / 8 Card / Gloss",
"price": "0.00",
"sku": "",
"position": 11,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Carbon Fiber",
"option2": "8 Card",
"option3": "Gloss",
"created_at": "2015-08-25T22:02:16-07:00",
"updated_at": "2015-08-29T21:36:42-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 997,
"old_inventory_quantity": 997,
"image_id": 4645790467,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6540945859,
"product_id": 2279964611,
"title": "Carbon Fiber / 12 Card / Gloss",
"price": "0.00",
"sku": "",
"position": 12,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Carbon Fiber",
"option2": "12 Card",
"option3": "Gloss",
"created_at": "2015-08-25T22:02:16-07:00",
"updated_at": "2015-09-01T16:40:13-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 997,
"old_inventory_quantity": 997,
"image_id": 4645790659,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6540945987,
"product_id": 2279964611,
"title": "Nickel / 4 Card / Polished",
"price": "0.00",
"sku": "",
"position": 13,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Nickel",
"option2": "4 Card",
"option3": "Polished",
"created_at": "2015-08-25T22:02:16-07:00",
"updated_at": "2015-09-01T22:43:46-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 994,
"old_inventory_quantity": 994,
"image_id": 4645785091,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6540945923,
"product_id": 2279964611,
"title": "Nickel / 8 Card / Polished",
"price": "0.00",
"sku": "",
"position": 14,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Nickel",
"option2": "8 Card",
"option3": "Polished",
"created_at": "2015-08-25T22:02:16-07:00",
"updated_at": "2015-09-02T09:38:16-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 979,
"old_inventory_quantity": 979,
"image_id": 4645787139,
"weight": 0,
"weight_unit": "lb"
}, {
"id": 6540946051,
"product_id": 2279964611,
"title": "Nickel / 12 Card / Polished",
"price": "0.00",
"sku": "",
"position": 15,
"grams": 0,
"inventory_policy": "continue",
"compare_at_price": null,
"fulfillment_service": "manual",
"inventory_management": null,
"option1": "Nickel",
"option2": "12 Card",
"option3": "Polished",
"created_at": "2015-08-25T22:02:16-07:00",
"updated_at": "2015-09-02T09:38:16-07:00",
"requires_shipping": true,
"taxable": true,
"barcode": "",
"inventory_quantity": 982,
"old_inventory_quantity": 982,
"image_id": 4645788547,
"weight": 0,
"weight_unit": "lb"
}],
"options": [{
"id": 2748080771,
"product_id": 2279964611,
"name": "Material",
"position": 1,
"values": [
"Titanium",
"Carbon Fiber",
"Nickel"
]
}, {
"id": 2748080835,
"product_id": 2279964611,
"name": "Size",
"position": 2,
"values": [
"4 Card",
"8 Card",
"12 Card"
]
}, {
"id": 2748080963,
"product_id": 2279964611,
"name": "Finish",
"position": 3,
"values": [
"Brushed",
"Stonewashed",
"Ti Black",
"Gloss",
"Polished"
]
}],
"images": [{
"id": 4645785091,
"product_id": 2279964611,
"position": 1,
"created_at": "2015-08-25T22:02:17-07:00",
"updated_at": "2015-08-25T22:02:17-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/Ni4.png?v=1440565337",
"variant_ids": [
6540945987
]
}, {
"id": 4645787139,
"product_id": 2279964611,
"position": 2,
"created_at": "2015-08-25T22:02:22-07:00",
"updated_at": "2015-08-25T22:02:22-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/Ni8.png?v=1440565342",
"variant_ids": [
6540945923
]
}, {
"id": 4645788547,
"product_id": 2279964611,
"position": 3,
"created_at": "2015-08-25T22:02:23-07:00",
"updated_at": "2015-08-25T22:02:23-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/Ni12.png?v=1440565343",
"variant_ids": [
6540946051
]
}, {
"id": 4645789059,
"product_id": 2279964611,
"position": 4,
"created_at": "2015-08-25T22:02:24-07:00",
"updated_at": "2015-08-25T22:02:24-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/Ti4.png?v=1440565344",
"variant_ids": [
6540945539,
6541017667,
6541044163
]
}, {
"id": 4645789379,
"product_id": 2279964611,
"position": 5,
"created_at": "2015-08-25T22:02:25-07:00",
"updated_at": "2015-08-25T22:02:25-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/Ti8.png?v=1440565345",
"variant_ids": [
6540945411,
6541017603,
6541044099
]
}, {
"id": 4645789635,
"product_id": 2279964611,
"position": 6,
"created_at": "2015-08-25T22:02:25-07:00",
"updated_at": "2015-08-25T22:02:25-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/Ti12.png?v=1440565345",
"variant_ids": [
6540945667,
6541017731,
6541044227
]
}, {
"id": 4645789827,
"product_id": 2279964611,
"position": 7,
"created_at": "2015-08-25T22:02:26-07:00",
"updated_at": "2015-08-25T22:02:26-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/C4.png?v=1440565346",
"variant_ids": [
6540945795
]
}, {
"id": 4645790467,
"product_id": 2279964611,
"position": 8,
"created_at": "2015-08-25T22:02:27-07:00",
"updated_at": "2015-08-25T22:02:27-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/C8.png?v=1440565347",
"variant_ids": [
6540945731
]
}, {
"id": 4645790659,
"product_id": 2279964611,
"position": 9,
"created_at": "2015-08-25T22:02:28-07:00",
"updated_at": "2015-08-25T22:02:28-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/C12.png?v=1440565348",
"variant_ids": [
6540945859
]
}],
"image": {
"id": 4645785091,
"product_id": 2279964611,
"position": 1,
"created_at": "2015-08-25T22:02:17-07:00",
"updated_at": "2015-08-25T22:02:17-07:00",
"src": "https://cdn.shopify.com/s/files/1/0898/7874/products/Ni4.png?v=1440565337",
"variant_ids": [
6540945987
]
}
}
}
];
}
function loadControls(){
//this is the control data
return [{
"product_id": 2280280195,
"variants-control": {
"6542377667": // No agenda included
{
"showConfigurator": false,
"restricted": true
},
"6542377731": // custom add on
{
"showConfigurator": true,
"restricted": false
},
"6542377795": // none
{
"showConfigurator": false,
"noPostOption": true,
"restricted": false,
"optOutAllowed": false
},
"6542377603": //custom included
{
"showConfigurator": true,
"restricted": true
},
"6633547075": // No Engraving - Carbon
{
"showConfigurator": false,
"noPostOption": true,
"restricted": true
}
}
},
{
"product_id": 2280223619,
"variants-control": {
"6541979011": //
{
"restricted": false,
"noPostOption":true
},
"6541978947": //
{
"restricted": false
},
"6544640067": //
{
"restricted": false
}
}
},
{
"product_id": 2280353923,
"variants-control": {
"6542749251": // Polished Stainless Steel
{
"selectNew": false,
"restricted": false
},
"6542749379": // Titanium - Add On
{
"selectNew": false,
"restricted": false
},
"6542749187": // None
{
"selectNew": true,
"noPostOption": true,
"restricted": false
},
"6542749443": // Polished Stainless Steel - Backer
{
"selectNew": false,
"restricted": true
},
"6542749507": // Titanium - Backer
{
"selectNew": false,
"restricted": true
}
}
}
];
}
var productReference = function (){
if(!_) {
throw new Error("you must have lodash!");
return;
}
console.log ('let\'s merge');
var controlData = loadControls();
var productData = loadProducts();
for (var i=0; i < controlData.length;i++) {
//roll through each controls set.
for (var j=0; j< productData.length ; j++) {
// clip
// wallet
if (productData[j].product.id == controlData[i].product_id){
var thisProductVariantsIds = Object.keys(controlData[i]["variants-control"]);
for (var x=0; x < productData[j].product.variants.length; x++) {
thisProductVariantsIds.forEach(function(item, index){
if ( productData[j].product.variants[x].id == item){
//WOOT!
_.defaults(productData[j].product.variants[x],controlData[i]["variants-control"][item]);
}
});
}
}
}
}
var out =[];
for( var i=0; i<productData.length; i++) {
switch(productData[i].product.id){
case 2280280195:
out.push({engravingVariants:productData[i].product});
break;
case 2280223619:
out.push({rfidBlockerVariants:productData[i].product});
break;
case 2280353923:
out.push({moneyClipVariants:productData[i].product});
break;
case 2279964611:
out.push({baseUnitVariants:productData[i].product});
break;
default:
console.log ('unfound option');
}
}
return out;
}();<file_sep>/assets/obrary.manager.js
(function (obraryManager, $, undefined) {
//Private Property
var productConfiguration;
var configuratorSource;
var resolvedConfiguratorOptions;
var defaultConfiguredItemInjector = function(snapshot){
// NOTE: this configured item injector does not support multiple items!
function getOtherShopifyFormLineItemProperties(){
var returnElements = [];
var propertiesMatchPattern = /properties\[([a-zA-Z0-9-\ ]+)\]/;
$(settingsData.product_form).serializeArray().forEach(function(formItem){
if (propertiesMatchPattern.test(formItem.name)){
formItem.name = formItem.name.replace(propertiesMatchPattern, function(str, p1){
return p1;
});
returnElements.push(formItem);
}
});
return returnElements;
}
if (snapshot){
var itemsToCartAdd = [];
shoppingResult = obraryManager.productData();
snapshot.items.forEach(function(lineItem, lineItemIdx){
var thisLineItem = {
id:shoppingResult.currentVariant.id,
quantity: lineItem.qty,
properties:{
'_snapshotId': snapshot.snapShotId + "/" + String(lineItemIdx+1)
}
};
snapshot.digest[lineItemIdx].attributes.forEach(function(attr){
// debugger;
console.log(attr);
thisLineItem.properties[attr.name] = [
"'" + attr.value + "'",
attr.type ==="String" ? "in font: " + attr.fontFace : ""
].join(" ");
});
getOtherShopifyFormLineItemProperties().forEach(function(formItem){
if (Object.keys(thisLineItem.properties).indexOf(formItem.name)===-1){
thisLineItem.properties[formItem.name] = formItem.value;
}
});
//now we add it to the
itemsToCartAdd.push(thisLineItem);
});
try{
obraryManager.doCustomCartAdd(itemsToCartAdd);
}
catch(err){
console.error("there was a problem adding the item to the cart", err);
}
}
else{
var errNoSnapshot =new Error("there should be a snapshot if the configured item injector is invoked");
console.error(errNoSnapshot.message);
//no error is thrown as the current time.
}
};
var configuratorVariantOption;
var configuratorVariantValue;
var cartAddDestination = "/cart";
var displayModeNarrow = false;
var listOfTests = {'productDataGood': undefined, 'requiredElements': undefined, 'injector': undefined};
var quantityControlEnabled = false;
var thisProduct;
var thisVariant;
var isPreFlightChecked = false;
var isInitialized = false;
console.log('Obrary Manager is being created');
//Private Method
function handleError(err) {
throw err;
}
//Private Method
function testAndCacheElements(presentedEls) {
//verify that all required elements are present.
var requiredEls = [];
var optionalEls = [];
//separate the list into
listOfCacheableElements.forEach(function (item, idx, arr) {
if (item.optional === false) {
requiredEls.push(item.name);
}
else if (item.optional === true) {
//the exclusive case to optional being true (loosely)
optionalEls.push(item.name);
}
else {
//these are undefined; no data defining mandatory/optional
}
});
var listofElementsProvided = Object.keys(presentedEls);
var testResult = listofElementsProvided.map(function (item, idx, arr) {
var hitTest = false;
for (var i = 0; i < requiredEls.length; i++) {
if (item === requiredEls[i]) {
hitTest = true;
var $testEl = $(presentedEls[requiredEls[i]]);
break;
}
}
return {
element: item, present: hitTest, elCount: function () {
if (hitTest === false) {
return 0
}
else {
return $testEl.length;
}
}()
};
});
var numThatAreValid = 0;
for (var i = 0; i < testResult.length; i++) {
if (testResult[i].present && testResult[i].elCount == 1) {
numThatAreValid++;
//We have passed the test(s), proceed to cache the element on the obrary manager object
obraryManager['$' + testResult[i].element] = $(presentedEls[testResult[i].element]);
if (!obraryManager.cachedEls){
obraryManager.cachedEls = {};
}
obraryManager.cachedEls['$' + testResult[i].element] = $(presentedEls[testResult[i].element]);
}
}
if (numThatAreValid == requiredEls.length) {
//We have passed the battery of tests
listOfTests.requiredElements = true;
}
else {
//we have failed some of the required elements
handleError("we have failed some of the required elements tests");
listOfTests.requiredElements = false;
}
//now go through the list of optional elements, and add them if possible
optionalEls.forEach(function (item, idx, arr) {
if ($(presentedEls[item])) {
obraryManager['$' + item] = $(presentedEls[item]);
}
//obraryManager['$'+ testResult[i].element]= $(presentedEls[testResult[i].element]);
});
}
function testProductData(product) {
//superficially test where there is product data
if (product) {
//pass the test
listOfTests.productDataGood = true;
//set the internal reference
thisProduct = product;
}
else {
listOfTests.productDataGood = false;
}
}
function runPreFlight() {
var tests = Object.keys(listOfTests);
var passes = 0;
for (var i = 0; i < tests.length; i++) {
if (listOfTests[tests[i]] === true) passes++;
}
if (passes == tests.length) {
isPreFlightChecked = true;
//isInitialized = true;
}
}
function bindInjector(inj, $configuratorRoot) {
if (typeof inj !== 'function') {
console.warn("A configuredItemInjector was not provided, attempting to use the default injector");
//handleError("the configured item injector must be a function");
$(settingsData.configurator_root)[0].configuredItemInjector = defaultConfiguredItemInjector;
listOfTests.injector = true;
}
else {
listOfTests.injector = true;
$(settingsData.configurator_root)[0].configuredItemInjector = inj;
}
}
function bindQuantityControl(settingsData) {
// Set the internal reference for whether external quantity control is enabled
quantityControlEnabled = settingsData.product_custom_quantity_enable;
$quantityControl = $(settingsData.quantity_control);
// Conditionally bind the quantity Control if it's enabled in the theme settings
if (quantityControlEnabled === true) {
$quantityControl.on('change', function () {
var $thisControl = $(this);
if (isInitialized === true) {
//console.log ('manager initialized and detected actionable event');
obraryManager.configurator.setItemQuantity(parseInt($thisControl.val()));
}
else {
//console.log('obrary detected that the quanity has changes but isn\'t going to do anything about it');
}
});
}
}
function bindVisibilityControl(settingsData) {
configuratorVariantOption = {
name: settingsData.product_custom_variant_dimension_name,
index: undefined
};
configuratorVariantValue = settingsData.product_custom_variant_keyword_value;
var hitOnOption = false;
thisProduct.options.forEach(function (item, idx, arr) {
if (item == configuratorVariantOption.name) {
//denote index position of the variant dimension
configuratorVariantOption.index = idx;
hitOnOption = true;
}
});
if (hitOnOption === false) {
handleError("The Variant Option used to control the configurator visility could not be found. Please check the settings in the Theme Control Panels");
}
}
function bindAdd2CartControl(settingsData) {
// Note:
// This is required since we disable the theme's standard add to cart button.
// It is changed in this implmentation to a div so that we can prevent default form post actions and handle the serialization
//bind the manager's doStandardCartAdd function
$(settingsData.add_to_cart_button_id).on('click', obraryManager.doStandardCartAdd);
//conditionally if this is pre-order bind that to the standard event handlers as well;
if ($(settingsData.add_to_preorder_button_id))
$(settingsData.add_to_preorder_button_id).on('click', obraryManager.doStandardCartAdd);
}
// Public method exposing Q.js based utility function for managing concurrence across multiple promises.
obraryManager.Throttle = function (values, max, iterator) {
max = max - 1;
var deferred = Q.defer();
var list = _.clone(values).reverse();
var outstanding = 0;
function catchingFunction(value) {
deferred.notify(value);
outstanding--;
if (list.length) {
outstanding++;
iterator(list.pop())
.then(catchingFunction)
.fail(rejectFunction);
}
else if (outstanding === 0) {
deferred.resolve();
}
}
function rejectFunction(err) {
deferred.reject(err);
}
while (max-- && list.length) {
iterator(list.pop())
.then(catchingFunction)
.fail(rejectFunction);
outstanding++;
}
return deferred.promise;
};
//public method that retrieves a copy of the product data
obraryManager.productData = function () {
if (isInitialized === true) {
return {reference: thisProduct, currentVariant: thisVariant};
}
else {
handleError("cannot return product data until initiation is complete");
}
};
obraryManager.currentOptions = function(){
return resolvedConfiguratorOptions;
};
// public method to initialize the manager (not the configurator)
obraryManager.initialize = function (injector, settingsData, productData, productMeta, configuratorOptions) {
// 1) bootstrap the control elements
// testAndCacheElements(criticalPageElements);
this.settingsData = settingsData;
// 2) take a copy of the product data in case it's needed by the configurator
testProductData(productData);
// 3) Run pre-flight checks.
runPreFlight();
// 4) ensure that there is a valid configuredItemInjector
bindInjector(injector, $(settingsData.configurator_root));
// 5) bind the quantity control from the page
bindQuantityControl(settingsData);
// 6) bind the visibility
if (!configuratorOptions.alwaysOn){
configuratorOptions.visible=true;
bindVisibilityControl(settingsData);
}
// 7) bind the add to page's cart button
bindAdd2CartControl(settingsData);
// 8) bootraph the configurator's basics
productConfiguration = productMeta.configuration_id;
configuratorSource = settingsData.product_configurator_source;
var defaultOptions = {
alwaysOn: false,
suppressVisualizationForUserAgents: true,
itemQuantity: obraryManager.getInitialQuantityValue(settingsData),
visible: configuratorOptions.alwaysOn ? true: false, //obraryManager.$visibilityControl.val(),
uniqueItemsMode: false, //this is a manager option to be non unique by default.
visualizerDocked: "true",
inputLocationStyle: "dock-right",
actionbutton: {
style: {},
class: $(settingsData.add_to_cart_button_id)[0].classList,
text: obraryManager.getTextForConfiguratorButton(settingsData),
enabled: true
},
visualization: {
type: 'default'
}
//displaydebugging: true,
//displaytools: false,
};
//set the default on the input options
_.defaultsDeep(configuratorOptions, defaultOptions);
//now that the values are set
resolvedConfiguratorOptions = configuratorOptions;
obraryManager.configurator = new obrary.Configurator($(settingsData.configurator_root), configuratorSource, productConfiguration, configuratorOptions);
isInitialized = true;
};
//public method to respond to select change events
obraryManager.selectChangeEvent = function (variant, selector, settingsData) {
//Note: this function implements the visibility control over which add to cart button is visible
if (isInitialized !== true) {
console.log('obrary detected an external change event, but has not initialized the configurator');
}
else {
thisVariant = variant;
console.log('obrary detected an external change event and the manager is initialized');
var newVisibilityState = obraryManager.currentOptions().alwaysOn ? true : variant.options[configuratorVariantOption.index] == configuratorVariantValue
obraryManager.configurator.setVisibility(newVisibilityState);
if (newVisibilityState === true) {
// debugger;
//hide the standard add to cart button
$(settingsData.add_to_cart_button_id).hide();
//
if ($(settingsData.add_to_preorder_button_id).length) {
$(settingsData.add_to_preorder_button_id).hide()
}
if ($(settingsData.tickle_panel)) {
$(settingsData.tickle_panel).hide().removeClass('fade-in').fadeIn(250);
}
}
else {
// the configurator is not visible
// debugger;
if ($(settingsData.tickle_panel)){
$(settingsData.tickle_panel).hide();
}
$(settingsData.gallery_thumbs).show();
if ($(this.settingsData.add_to_preorder_button_id).length) {
$(this.settingsData.add_to_preorder_button_id).show();
$(this.settingsData.add_to_cart_button_id).hide();
} else {
$(this.settingsData.add_to_cart_button_id).hide().removeClass('fade-in').fadeIn(250);
}
}
}
};
obraryManager.getInitialQuantityValue = function (settingsData) {
if (quantityControlEnabled === true) {
return Number($(settingsData.quantity_control).val());
}
else {
return 1;
}
};
//public method to post a customized item to the cart
obraryManager.doCustomCartAdd = function (items, retryLimit, navigateAway) {
//critical stuff
var deferred = $.Deferred();
var cartUrl ='/cart/add';
var results = {errors: [], successes: []};
//flip the order of the array so that they end up in the cart first...last
items.reverse();
//set some defaults not provided
navigateAway = navigateAway ===false ? undefined: cartAddDestination;
retryLimit = retryLimit || 3;
//resolving the final disposition of the completed cart post action
function wrapUp() {
if (!results.errors.length) {
deferred.resolve(true)
if (navigateAway){
window.location = navigateAway;
}
}
else {
var terminalError = new Error("some items could not be processed");
terminalError.detail = results.errors;
deferred.reject(terminalError);
//todo change configurator state to error condition.
obraryManager.configurator.$rootScope.configuratorCtlr.setConfiguratorActionState("failed");
}
}
//the main work unit
function processQueue(unit, curRetry) {
thisItem = items[unit];
try {
if (curRetry <= retryLimit) {
//setup the options object with the data we would like to post
var options = {
dataType: 'JSON',
type: "POST",
data: thisItem
};
$.ajax(cartUrl, options)
.then(
//post success
function () {
//pop the item off of the stack
console.log("cartPost: individual item success!");
results.successes.push(items.pop());
if (items.length) {
processQueue(items.length - 1, 1);
}
else {
wrapUp();
}
},
//post failure
function (e) {
//failure, let's retry if it's not a 404
if (unit >= 0) {
if (e.status !== 404) {
setTimeout(function () {
processQueue(unit, curRetry + 1)
}, e.status === 429 ? 5000 : 400);
}
else {
//continue recursion with a value we know won't process
processQueue(unit, retryLimit + 1)
}
}
else {
wrapUp();
}
});
}
else {
//we hit retry limit, so we're moving on the next unit
results.errors.push(items.pop());
processQueue(unit - 1, 1)
}
}
catch (e) {
//catchall error trap
deferred.reject(e);
}
}
//execute the recursion
processQueue(items.length - 1, 1);
return deferred;
};
//public method to reconfigure a standard post (obrary configurator changes standar from HTML button to div to prevent loose javascript from posting cart items)
obraryManager.doStandardCartAdd = function () {
console.log('add to cart for standard form requested...');
var postSettings = {
success: function (result) {
window.location = cartAddDestination;
},
error: function (err) {
console.error("There was an Error processing the Cart Addition");
},
type: $(obraryManager.settingsData.product_form).attr('method'),
data: $(obraryManager.settingsData.product_form).serialize()
};
$.ajax($(obraryManager.settingsData.product_form).attr('action'), postSettings);
};
obraryManager.getTextForConfiguratorButton = function () {
if ($(obraryManager.settingsData.add_to_preorder_button_id).length) {
//use the text from the pre order control
return $(obraryManager.settingsData.add_to_preorder_button_id).children().first().text();
}
else {
//use the standard button text
return $(obraryManager.settingsData.add_to_cart_button_id).children().first().text();
}
};
})(window.obraryManager = window.obraryManager || {}, jQuery); | 126187d56f29e6a9f31a4e2ab9a2b73d1b827649 | [
"JavaScript"
] | 2 | JavaScript | inseev/etchingexpressions | f65ee0fa621eff8d0cbbbdd33845616dd41a7713 | 5e3ae9f64e3d8fad81c4e4dab1912bff3383e441 |
refs/heads/master | <file_sep>import * as _ from 'lodash';
import * as d3 from 'd3';
// TODO
// parameterize this
export const transformMeltedToNestedCategorical = function (props){
let categories = _.uniqBy(_.map(props.data, props.category));
props.data = _.map(categories, (currentVar) =>{
return {
category: currentVar,
values: _.map(_.filter(props.data, d => props.category(d) === currentVar),
d => ({ x: +props.x(d), y: +props.y(d)}))
}
});
props.x = d => d.x;
props.y = d => d.y;
props.values = d => d.values;
props.category = d => d.category;
return props;
}
/**
* Draws simple X-Y axis
*/
export const drawXYAxes = function (props, selection){
props.xAxis = props.xAxis || d3.axisBottom;
props.yAxis = props.yAxis || d3.axisLeft;
// Axes
let xAxis = props.xAxis(props.xScale).ticks(5).tickSizeOuter([0]);
let yAxis = props.yAxis(props.yScale).ticks(5).tickSizeOuter([0]);
// create <g> to hold axes
let gX = selection.select(`.x-axis`);
if(gX.empty()){
gX = selection.append('g').classed('x-axis', true);
}
let gY = selection.select(`.y-axis`);
if(gY.empty()){
gY = selection.append('g').classed('y-axis', true);
}
gX.transition().duration(700).call(xAxis);
gY.transition().duration(700).call(yAxis);
// move x axis to bottom
gX.attr('transform', `translate(0, ${props.height + 10})`);
gY.attr('transform', 'translate(-10, 0)');
return props;
}
/**
* Draws a multiseries set of lines
* grouped by categorical data
*/
export const drawCategoricalPaths = function(props, selection){
// draw lines for dleata
let pathfn = d3.line().curve(d3.curveBasis)
.x(d => props.xScale(props.x(d)))
.y(d => props.yScale(props.y(d)));
let paths = selection.selectAll('path')
.data(props.data);
paths.exit()
.remove();
paths.transition()
.duration(700)
.ease(d3.easeLinear)
.attr('d', d => pathfn(props.values(d)))
paths.enter()
.append('path')
.attr('d', d => pathfn(props.values(d)))
.attr('stroke', 'yellow')
.attr('stroke-width', '2px')
.attr('fill', 'none')
.on('mouseover', function(){
d3.select(this)
.transition()
.duration(300)
.ease(d3.easeLinear)
.attr('stroke-width', '6px');
})
.on('mouseout', function(){
d3.select(this)
.transition()
.duration(300)
.ease(d3.easeLinear)
.attr('stroke-width', '2px');
});
return props;
}<file_sep># Charting Library
This charting library is more of a reusable framework for d3 charts rather than a comprehensive library for data visualizations. It is inspired by the seminal paper "Grammar of Graphics" by <NAME>, and of course <NAME>'s "A Layered Grammar of Graphics".
Or for those who know R, this is a javascript version of GGPlot2 with events!
## Wait, Grammar of Graphics what?
In his original paper, Wilkinson set out to establish a "grammar" for data visualizations, the bare minimum parameters you need to define to convert a dataset into a visualization.
For starters, you need data. This data will map on to geometric shapes on the plot, for example, lines in a linegraph and points in a scatterplot or nodes/edges in a graph. Now we need a definition for how the data should be mapped to the geometric shape. This could just be how the position of the geometric shapes maps to the data, or it could be other aesthetic features such as size and color. Now we also need a scale to scale the data to pixels on the screen to make sure all the data fits in. We can also add in data transformations, for when we want to plot a frequency/distribution plot, such as a histogram. Lastly, we can also add facets, which I'll talk about later.
For example, if we have population data for people of different income groups in a city over the years, we have three columns, Year, Population and Income Group, and we want to draw a linegraph. Here, we can define Year to the x-coordinate of line and Population to the y-coordinate of of the line and we have a simple linegraph. We can also now provide a color aesthetic to map different colors to the lines based on the income group. Easy!
So here is what the grammar of graphics as proposed by Wilkinson:
* Data
* Aesthetic Mappings
* Geometry
* Scale
* Data Transforms
* Facets
Now <NAME>, in his paper, proposed a layered grammar where a given visualization has multiple layers, and each layer could have it's own data, aesthetics, geomety, scale, transforms and facets. Even better!
[Here's](http://byrneslab.net/classes/biol607/readings/wickham_layered-grammar.pdf) a link to Wickham's paper. I couldn't find Wilkinson's original paper for free online but I recommend reading it as well for a deeper understanding of the subject.
## Why?
As I was working on a visualization project recently, I found that writing reusable D3 code is not easy or straightforward. There is an elegant way proposed by the phenomenal Mike Bostock(creator of D3), as outlined [here](https://bost.ocks.org/mike/chart/). It makes clever use of the cascading pattern and is simply elegant.
However, it does have its limitations in certain use cases. For example, lets say you made a reusable linegraph according to Mike's pattern. Now let's say you wanted to add a scatterplot to the linegraph. You would have to internally modify the original code, or worse, copy the original code and make a separate module that now draws a linegraph along with a scatterplot.
This is something that can easily be solved using a Grammar of Graphics approach. For the linegraph you pass in the data, scale and aesthetics. Now to add a scatterplot, now you can just add another layer that shares the same data and scale, and aesthetics. The only thing that changes is the geometry for the new layer.
Using a GoG approach, as in GGPlot2 or my framework, this is how we'd do it:
Let's say we have a function that draws categorical paths(paths colored according to their category), called drawCategoricalPaths, and another function called drawScatter that draws simple points based on category as well.
We can create a Layer by passing in a 'props' object that is used to initialize layer properties.
for the linegraph, we only need to define the data, its aesthetic mappings(x, y, category) and geometry.
```javascript
let linegraph = Layer({
data: data,
x: d => d.Time,
y: d => d.value,
category: d => d.variable,
geom: [drawCategoricalPaths],
//...other aesthetic mappings such as height, width, margins etc
});
```
now to transform to a linegraph with scatterplot:
```javascript
let scatterplot = Layer({
data: data,
x: d => d.Time,
y: d => d.value,
category: d => d.variable,
geom: [drawScatter],
//...other aesthetic mappings such as height, width, margins etc
});
let linegraphWithScatterPlot = new LayerGroup([linegraph, scatterplot])
```
To add more stuff the the graph, all you need to do is add more layers. Maximum code reuse!
In fact you don't even need to define a separate layer for adding the scatterplot to the original linegraph. That was done to show the use case for more complicated examples, but for this particular example, you can simply modify the original definition of linegraph as follows:
```javascript
let linegraph = Layer({
data: data,
x: d => d.Time,
y: d => d.value,
category: d => d.variable,
geom: [drawCategoricalPaths, drawScatter], // <- just add drawScatter to the list of geometries!
//...other aesthetic mappings such as height, width, margins etc
});
```
Now you will need to have coded drawCategoricalPaths and drawScatter, but you don't have to worry about whether these two functions will "mess" with each other. All you need to know is you will be given an SVG <g> element, and you need to draw on it. [Feature Not Complete] You can define dependencies, for example, that your draw function needs an x aesthetic and a y aesthetic, and also some data!
Lastly, this framework uses a pub/sub event pattern, where each layer can have its own events. This provides a lot of room for composition.
#### To summarize, this is an alternative reusable framework for writing reusable D3 charts
# Architecture
## Basic Components of a Chart, GoG style
#### Essential Properties: Data, Aesthetics, Geometries, Scales and Data Transforms
These are the basic building blocks of any layer of a graph described in the GoG style
#### Layers
A *Layer* is the smallest unit of the GoG universe that can be rendered by itself, so long as it's *essential properties* have been defined. The *Layer* handles the actual rendering of the plot; it performs the data transform and the scaling, then calls on the geometry function to actually plot the graph. The *Layer* also handles events and updates, which will be discussed later on.
#### LayerGroup
A *LayerGroup*, as the name suggests, is basically a bunch of *Layers*. It supports all operations that a single *Layer* can perform. Instead of having a separate chart wrapper that contains *Layers* within it, I simply made a *LayerGroup* to keep the API simple and uniform.
## Events and Updates
This library uses a *pub/sub* model for events. Any DOM event that exists, you can subscribe to it. But how does this work across *Layers*?
Well, you are able to subscribe to events on individual *Layers*, but you can also subscribe to events on a particular element inside a particular geometry. We need to get into the details to understand this.
Each geometry can register elements that are available for events. So in the earlier lineplot with scatter points example, the line geometry component can register *both* or either the lines or the scatter points. As a user of this geometry, you can then subscribe to whichever elements you need. You can do this either by reading the specific documentation for the geometry to figure out which events are available, or ask the *Layer* for a list of all geometries and events supported by them.
The reason for this system is that each geometry can be unique and can have multiple elements to it that might be relevant to certain operations, and its hard to enumerate and account for all possibilities preemptively. The current system frees the geometry creator of having to think about how events will be used by users of the framework. They can just register as many or as few elements as they like and its up to the user of the geometry to decide which elements they want to listen to and which to ignore,
The updates work in similar fashion, however updates work on geometry level rather than for individual elements of a geometry. A geometry creator can define the what can be updated, and the actual logic of the update. On the other side, the user can trigger the update with the new property and not have to worry about how the update is actually propogated.
<file_sep>import * as _ from 'lodash';
import * as d3 from 'd3';
import {Layer} from '../../core/Layer'
import {drawXYAxes, drawCategoricalPaths} from './draw-functions';
function subscriber(fn, updateEventName){
return function(props, selection){
console.log("inside")
fn(props, selection);
props.mediator.subscribe(updateEventName, function(updatedProps){
return fn(updatedProps, selection);
});
return props;
}
};
function MultiseriesLinegraph(props){
function chart(){
let layer = Layer({
x: props.x,
y: props.y,
category: props.category,
dataTransform: transformMeltedToNestedCategorical,
height: props.height,
width: props.width,
color: d => d.color,
geom: [subscriber(drawCategoricalPaths, "dataUpdate")],
axes: subscriber(drawXYAxes, "dataUpdate")
});
}
chart.draw = function(selection){
}
}<file_sep>
export const compose = (f, g) => {
return function (...args) {
return f(g(...args));
}
}
export const curry = (fn) => {
return function curriedFn(...args){
if(args.length < fn.length){
return (...rest) => curriedFn(...(rest.concat(args)));
}else{
return fn(...args);
}
}
}
/**
* given the props object, the attribute name and an
* update function for that attribute, creates a mutator
* function
*/
export const createMutator = function(props, propName, updateFn, value){
console.log("createMutator");
return function (...args){
if(value){
// console.log(typeof updateFn);
if(props[propName] && typeof updateFn === 'function'){
props[propName] = args[0];
updateFn(props);
}else{
props[propName] = args[0];
}
return this;
}else{
return props[propName];
}
}
}
/**
* Monads and Gonads
*/
export const Once = (fn) => {
let flag = true;
return function(...args){
if(flag){
flag = false;
return fn(...args);
}
}
}
export const MONAD = function(modifier){
let prototype = Object.create(null);
function unit(value){
let monad = Object.create(prototype);
monad.bind = function(fn, ...args){
return fn(value, ...args)
}
if(typeof modifier === 'function'){
modifier(monad, value);
}
return monad;
}
unit.lift = function(name, fn){
prototype[name] = function(...args){
return unit(this.bind(fn, ...args))
}
}
return unit;
}
export const Maybe = MONAD(function(monad, value){
if(value === null || value === undefined){
monad.is_null = true;
monad.bind = function(){
return monad;
}
}
});
<file_sep>import * as _ from 'lodash';
import * as d3 from 'd3';
import * as d3Hsv from 'd3-hsv'
import * as d3Contours from 'd3-contour';
require('d3-shape');
require('d3-scale');
require('d3-transition');
require('d3-contour');
import {basicMarginInitializer} from '../common/init-functions';
export const heatmapGridInitializer = function(props){
let maxCols = d3.extent(props.data, props.x)[1];
let maxRows = d3.extent(props.data, props.y)[1] + 1;
props = basicMarginInitializer(props)
console.assert(props.height > 0, "invalid height or margin: " + props.height);
console.assert(props.width > 0, "invalid width or margin: ");
props.gridHeight = (props.height / maxRows);
props.gridWidth = props.width / maxCols;
props.numberOfCols = maxCols;
props.numberOfRows = maxRows;
return props;
}
export const drawContours = function(props, selection){
let heatmapValues = {};
props.data.forEach(d => {
let row = props.y(d), col = props.x(d);
if(heatmapValues[row] === null || heatmapValues[row] === undefined
|| heatmapValues[row][col] === null || heatmapValues[row][col] === undefined){
heatmapValues[row] = heatmapValues[row] || [];
heatmapValues[row][col] = props.z(d);
if(props.z(d) > 0)
console.log(`(${row}, ${col} => ${props.z(d)})`)
}
});
let heatmapFlattenedArray = [];
for(let row = 0; row < props.numberOfRows; row++){
for(let col = 0; col < props.numberOfCols; col++){
if(heatmapValues[row] && heatmapValues[row][col]){
heatmapFlattenedArray.push(heatmapValues[row][col]);
}else{
heatmapFlattenedArray.push(0.0);
}
}
}
// let n = props.numberOfCols, m = props.numberOfRows, values = new Array(n * m);
// for (let j = 0, k = 0; j < m; ++j) {
// for (let i = 0; i < n; ++i, ++k) {
// values[k] = heatmapValues[j][i];
// }
// }
// console.log(values);
// heatmapFlattenedArray = values;
let extent = d3.extent(heatmapFlattenedArray);
// heatmapFlattenedArray.forEach((d, i) => console.log(`${i}: ${d}`))
let i0 = d3Hsv.interpolateHsvLong(d3Hsv.hsv(120, 1, 0.65), d3Hsv.hsv(60, 1, 0.90)),
i1 = d3Hsv.interpolateHsvLong(d3Hsv.hsv(60, 1, 0.90), d3Hsv.hsv(0, 0, 0.95)),
interpolateTerrain = function(t) { return t < 0.5 ? i0(t * 2) : i1((t - 0.5) * 2); },
color = d3.scaleSequential(interpolateTerrain).domain(extent);
let contourData = d3Contours.contours()
.size([props.numberOfCols, props.numberOfRows])
.thresholds(d3.range(...extent, 0.01))(heatmapFlattenedArray);
// let height = props.numberOfRows * props.gridHeight;
// let width = props.numberOfCols * props.gridWidth;
let scalingFactor = Math.max(props.gridHeight,
props.gridWidth)
selection.selectAll("path")
.data(contourData)
.enter().append("path")
.attr("d", d3.geoPath(d3.geoIdentity().scale(scalingFactor)))
.attr("fill", d => color(d.value));
}
/**
* requires: [props.text, props.x, props.y, props.z, props.gridHeight, props.gridWidth]
* optional: font-size
*/
export const drawText = function (props, selection, heatmapClassName = 'heatmap-text'){
let heatmapText = selection.select(`.${heatmapClassName}`);
if(heatmapText.empty()){
heatmapText = selection.append("g")
heatmapText.classed('${heatmapClassName}');
}
let elemRoot = heatmapText.selectAll('text').data(props.data);
elemRoot.enter().append('text')
.attr('x', (d, i) => +props.x(d) * props.gridWidth + (props.gridWidth / 2))
.attr('y', (d, i) => (+props.y(d) + 1) * props.gridHeight - (props.gridHeight / 2))
.text(props.text)
.attr('text-anchor', 'middle')
.attr('alignment-baseline', 'middle')
.attr('font-size', Math.min(props.gridHeight, props.gridWidth));
elemRoot.attr('x', (d, i) => +props.x(d) * props.gridWidth + (props.gridWidth / 2))
.attr('y', (d, i) => (+props.y(d) + 1) * props.gridHeight - (props.gridHeight / 2))
.text(props.text)
.attr('text-anchor', 'middle')
.attr('alignment-baseline', 'middle')
.attr('font-size', Math.min(props.gridHeight, props.gridWidth));
elemRoot.exit().remove();
}
// export const drawHeatmap = function(props, selection){
// let n = props.numberOfCols,
// m = props.numberOfRows;
// let canvas = selection.select("canvas")
// .attr("width", n)
// .attr("height", m);
// let context = canvas.node().getContext("2d"),
// image = context.createImageData(n, m);
// for (let j = 0, k = 0, l = 0; j < m; ++j) {
// for (let i = 0; i < n; ++i, ++k, l += 4) {
// let c = d3.rgb(color(volcano.values[k]));
// image.data[l + 0] = c.r;
// image.data[l + 1] = c.g;
// image.data[l + 2] = c.b;
// image.data[l + 3] = 255;
// }
// }
// context.putImageData(image, 0, 0);
// }
export const drawGrid = function(props, selection, gridClassName = 'grid'){
let grid = selection.select(`.${gridClassName}`);
if(grid.empty()){
grid = selection.append("g");
grid.classed(gridClassName, true);
}
let gridElems = grid.selectAll('rect')
.data(props.data);
gridElems.enter()
.append('rect')
.attr('x', (d, i) => (props.x(d) * props.gridWidth))
.attr('y', (d, i) => (props.y(d) * props.gridHeight))
.attr('height', props.gridHeight)
.attr('width', props.gridWidth)
.attr('stroke', 'black')
.attr('fill', d => {
if(props.color)
return props.color(d.duration)
else return 'none';
} || 'none' )
.attr('opacity', 0.9)
.on("click", d =>{
console.log(d);
});
}<file_sep>import {MONAD} from '../utilities/functionalUtils';
let Mediator = MONAD();
Mediator.lift("subscribe", function(eventRegistry, eventName, eventHandler){
if(eventRegistry && eventHandler){
if(eventRegistry[eventName] === null ||eventRegistry[eventName] === undefined){
eventRegistry[eventName] = []
}
eventRegistry[eventName].push(eventHandler);
}
return eventRegistry;
});
Mediator.lift("publish", function(eventRegistry, eventName, ...args){
if(eventRegistry && eventName){
let eventEntry = eventRegistry[eventName];
if(eventEntry){
eventEntry.forEach(function(eventHandler){
eventHandler(...args);
})
}else{
eventRegistry[eventName] = [eventName];
}
}
return eventRegistry;
});
export {Mediator}; | 7ec016f2406624bc3bb55d45d3ab9d2ac6fcd64d | [
"JavaScript",
"Markdown"
] | 6 | JavaScript | devjeetr/Charting-Library | db8185eec2b9e78bf587a19e616a5096cae38181 | b1c1ee087962a37bca462c6dba23f699c760fbb3 |
refs/heads/master | <file_sep><!DOCTYPE html>
<html lang="en">
<head>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8">
<title><NAME></title>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.min.js"></script>
<link rel="stylesheet" type="text/css" href="<?php echo esc_url( get_template_directory_uri() ); ?>/style.css">
<script src="//use.typekit.net/cqr7mtq.js"></script>
<script>try{Typekit.load();}catch(e){}</script>
<meta name=viewport content="width=device-width, initial-scale=1">
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.min.js"></script>
<?php wp_head(); ?>
</head>
<body style="-webkit-text-size-adjust: none; -ms-text-size-adjust: none; margin: 0; padding: 0;">
<div class="main">
<div class="centralw">
<div class="logo">
<a href="<?php echo site_url(); ?>"><img src="<?php echo esc_url( get_template_directory_uri() ); ?>/images/logo.jpg" alt="" style="display: block; border: 0" width="435"></a>
</div>
<div class="secondarymenu">
<?php
wp_nav_menu(array(
'theme_location' => 'header',
'menu' => '',
'container' => 'nav',
));
?>
</div>
</div>
<?php if (is_front_page()) : ?>
<div class="fullw">
<div class="header">
<div class="header-image-holder">
<img src="<?php echo esc_url( get_template_directory_uri() ); ?>/images/header.jpg" alt="" style="display: block; border: 0">
</div>
<div class="header-copy">
<p>Insurance Brokers & Independent Financial Advisers</p>
</div>
</div>
</div>
<?php else : ?>
<div class="fullw">
<div class="header-pages">
<div class="header-image-holder-pages">
<img src="<?php echo esc_url( get_template_directory_uri() ); ?>/images/header.jpg" alt="" style="display: block; border: 0">
</div>
<div class="header-copy">
<p>Insurance Brokers & Independent Financial Advisers</p>
</div>
</div>
</div>
<div class="fullw">
<div class="main-menu-holder-responsive">
<a class="burger" href="#">
<div class="burger-icon">
<span></span>
</div>
<span class="burger-label">Menu</span>
</a>
</div>
<div class="main-menu-holder">
<?php
wp_nav_menu(array(
'theme_location' => 'primary',
'menu' => '',
'container' => 'nav',
));
?>
</div>
</div>
<div class="centralw">
<?php get_template_part('breadcrumbs'); ?>
</div>
<?php Endif
?><file_sep>
<div class="fullw fullw-dark-grey">
<div class="footer-nav">
<?php
wp_nav_menu(array(
'theme_location' => 'footer',
'menu' => '',
'container' => 'nav',
));
?>
</div>
</div>
<div class="centralw">
<div class="footer-left">
<div class="footer-socail">
<a href="https://twitter.com/mwlinsurance" target="_blank"><img src="<?php echo esc_url( get_template_directory_uri() ); ?>/images/twitter.jpg" alt="" style="display: block; border: 0"></a>
<a href="https://www.linkedin.com/company/marshall-wooldridge-ltd?trk=company_logo" target="_blank"><img src="<?php echo esc_url( get_template_directory_uri() ); ?>/images/linkedin.jpg" alt="" style="display: block; border: 0"></a>
</div>
<div class="logos">
<img src="<?php echo esc_url( get_template_directory_uri() ); ?>/images/logos_bottom.jpg" alt="" style="display: block; border: 0">
</div>
</div>
<div class="footer-right">
<p>Marshall Wooldridge Ltd. Authorised and Regulated by the Financial Conduct Authority. Registration No. 136079. Registered Office: 14-16 Ivegate, Yeadon, Leeds, LS19 7RE. Registered in England No. 1093348. ©2015 Copyright.</p>
</div>
</div>
</div>
<?php wp_footer(); ?>
</body>
</html><file_sep><?php get_header(); ?>
<p>Hello im the blog!</p>
<?php get_footer(); ?><file_sep><?php
$globalid = get_the_ID();
$menuitems = new WP_Query( array(
'post_parent' => $globalid,
'post_type' => 'page',
'post_status' => 'publish',
'posts_per_page' => -1
) );?>
<div class="main-items-holder">
<?php
while ($menuitems->have_posts()) : $menuitems->the_post();
$icon = types_render_field('icon', array('url'=>'true'));
if ($icon == "") {
$icon = types_render_field('icon', array('post_id' => $globalid,'url'=>'true'));
}
?>
<!--[if IE 9]><div class="floatIE"><![endif]--><!--[if IE 8]><div class="floatIE"><![endif]-->
<div class="main-items-item">
<div class="main-items-item-img">
<div class="circle">
<img src="<?php echo $icon ?>" alt="" style="display: block; border: 0">
</div>
<div class="gradient-line"></div>
</div>
<div class="main-items-item-copy">
<h3><?php the_title(); ?></h3>
<p>
<?php the_excerpt(); ?>
<br>
<a href="<?php echo get_permalink(); ?>">[Read more]</a>
</p>
</div>
</div>
<!--[if IE 9]></div><![endif]--><!--[if IE 8]></div><![endif]-->
<?php
endwhile;
?>
</div>
<file_sep><?php
$chartered = new WP_Query(array(
'post_type' => 'page',
'post_status' => 'publish',
'pagename' => 'chartered-status',
'order' => 'DESC'
));
$callback = new WP_Query(array(
'post_type' => 'page',
'post_status' => 'publish',
'pagename' => 'request-a-callback',
'order' => 'DESC'
));
?>
<div class="light-grey-holder">
<?php
while ($chartered->have_posts()) : $chartered->the_post();
?>
<div class="grid-left">
<?php if (has_post_thumbnail()) : ?>
<?php the_post_thumbnail(); ?>
<?php else: ?>
<img src="<?php echo esc_url( get_template_directory_uri() ); ?>/images/backup.jpg" alt="" style="display: block; border: 0">
<?php endif; ?>
</div>
<div class="grid-middle">
<h3><?php the_title(); ?></h3>
<?php the_excerpt(); ?>
<a href="<?php the_permalink(); ?>">[Read more]</a></p>
</div>
<?php
endwhile;
?>
<?php
while ($callback->have_posts()) : $callback->the_post();
?>
<div class="grid-right">
<h3><?php the_title(); ?></h3>
<?php the_excerpt(); ?>
<div class="button"><a href="<?php echo site_url(); ?>/contact-us/">Request now</a></div>
</div>
<?php
endwhile;
?>
</div><file_sep>
<?php
/*
Template name: Landing Page
*/
get_header();
?>
<div class="centralw">
<?php get_template_part('teamplate-items-circle-child'); ?>
</div>
<div class="fullw fullw-light-grey">
<?php get_template_part('teamplate-chartered-callback'); ?>
</div>
<div class="centralw">
<?php get_template_part('teamplate-mini-blog'); ?>
</div>
<?php get_footer(); ?><file_sep><?php get_header(); ?>
<div class="centralw">
<?php get_template_part('teamplate-items-circle-parent'); ?>
</div>
<div class="fullw fullw-light-grey">
<?php get_template_part('teamplate-chartered-callback'); ?>
</div>
<div class="centralw">
<?php get_template_part('teamplate-mini-blog'); ?>
</div>
<?php get_footer(); ?><file_sep>
<?php
/*
Template name: About Page
*/
get_header();
?>
<?php
$users = new WP_User_Query(array(
'meta_key' => 'team',
'meta_value' => 1
));
?>
<?php
while (have_posts()) : the_post();
?>
<div class="centralw">
<div class="main-holder">
<div class="main-items-left">
<h2><?php the_title(); ?></h2>
<?php if (has_post_thumbnail()) : ?>
<div class="image-main"><?php the_post_thumbnail(); ?></div>
<?php else: ?>
<?php endif; ?>
<?php the_content(); ?>
</div>
<div class="main-items-right">
<h3>Meet the team</h3>
<div class="gradient-line"></div>
<div class="side-holder">
<?php
foreach ($users->results as $user) :
$image = get_avatar($user->ID, 100)
?>
<div class="side-memeber">
<div class="side-member-img">
<?php echo $image ?>
</div>
<h3><?php echo $user->first_name ?> <?php echo $user->last_name ?></h3>
<p><?php echo $user->job_title ?></p>
</div>
<?php
endforeach;
?>
</div>
<br>
<div class="button center"><a href="">See full details</a></div>
</div>
</div>
</div>
<div class="fullw fullw-light-grey">
<?php get_template_part('teamplate-contact-callback'); ?>
</div>
<div class="centralw">
<?php get_template_part('teamplate-mini-blog-cat'); ?>
</div>
<?php
endwhile;
?>
<?php get_footer(); ?><file_sep>
jQuery(document).ready(function(){
jQuery("a.burger").click(function(){
jQuery(this).parent().next().slideToggle(function(){
jQuery(this).toggleClass("menutotoggle");
});
jQuery(this).toggleClass("selected");
});
});
<file_sep><?php
$posts = new WP_Query(array(
'post_type' => 'post',
'post_status' => 'publish',
'orderby' => 'date',
'order' => 'DESC',
'posts_per_page' => 4
));
?>
<div class="news-advice-holder">
<h3>NEWS AND ADVICE</h3>
<?php
while ($posts->have_posts()) : $posts->the_post();
?>
<div class="new-advice-bloggy">
<div class="new-advice-bloggy-image">
<?php if (has_post_thumbnail()) : ?>
<a href=""><?php the_post_thumbnail(); ?></a>
<?php else: ?>
<a href=""><img src="<?php echo esc_url( get_template_directory_uri() ); ?>/images/backup.jpg" alt="" style="display: block; border: 0"></a>
<?php endif; ?>
<div class="little-date"><p><?php echo get_the_date(); ?></p></div>
</div>
<div class="new-advice-bloggy-copy">
<p><strong><a href=""><?php the_title(); ?></a></strong></p>
<p>By: <?php echo get_the_author(); ?></p>
</div>
<div class="button"><a href="<?php the_permalink(); ?>">Read full article</a></div>
</div>
<?php
endwhile;
?>
</div>
<file_sep><?php get_header(); ?>
<?php
while (have_posts()) : the_post();
?>
<div class="centralw">
<div class="main-holder">
<div class="main-items-left">
<h2><?php the_title(); ?></h2>
<?php if (has_post_thumbnail()) : ?>
<div class="image-main"><?php the_post_thumbnail(); ?></div>
<?php else: ?>
<?php endif; ?>
<?php the_content(); ?>
</div>
<div class="main-items-right">
<h3>testimonials</h3>
<div class="gradient-line"></div>
<div class="side-holder">
<div class="side-holder-testimonail">
<?php echo types_render_field('add-testimonial-copy', array('output' => 'raw', 'separator' => '<br><br>')); ?>
</div>
</div>
</div>
</div>
</div>
<div class="fullw fullw-light-grey">
<?php get_template_part('teamplate-contact-callback'); ?>
</div>
<div class="centralw">
<?php get_template_part('teamplate-mini-blog-cat'); ?>
</div>
<?php
endwhile;
?>
<?php get_footer(); ?><file_sep><?php
//setup
if (!function_exists('m_w_db_setup')){
function m_w_db_setup() {
add_theme_support( 'post-thumbnails' );
set_post_thumbnail_size( 960, 226, true );
add_image_size('homepage-about', 120, 236, true);
register_nav_menus(array(
'primary' => __('Primary Menu','m_w_db'),
'footer' => __('Footer Menu','m_w_db'),
'header' => __('Header Menu','m_w_db')
));
}
}
add_action('after_setup_theme','m_w_db_setup');
//add Javascript file
if (!function_exists('m_w_db_scripts')) {
function m_w_db_scripts() {
wp_enqueue_script( 'm_w_db-script', get_template_directory_uri() . '/script.js', array('jquery'), '20150427', true );
}
}
add_action('wp_enqueue_scripts','m_w_db_scripts');
//add widgets sidebar
if (!function_exists('m_w_db_widget')){
function m_w_db_widget() {
register_sidebar(array(
'name' => __('Right blog sidebar', 'm_w_db'),
'id' => 'right-blog-sidebar',
'description' => __('Sidebar on the right of the blog', 'm_w_db')
));
register_sidebar(array(
'name' => __('Search Bar', 'm_w_db'),
'id' => 'serach-bar',
'description' => __('Serach bar on top of each page', 'm_w_db')
));
}
}
add_action('widgets_init','m_w_db_widget');
//User profile
if (!function_exists('m_w_db_user_profile_show')) {
function m_w_db_user_profile_show($user) {
?>
<h3><?php echo __('Additional Informations', 'm_w_db') ?></h3>
<table class="form-table">
<tr>
<th><lable for="job-title">Job Title</lable></th>
<td><input id="job-title" type="text" name="job_title" value="<?php echo esc_attr(get_the_author_meta('job_title', $user->ID)); ?>" class="regular-text"></td>
</tr>
<tr>
<th><lable for="phone">Phone</lable></th>
<td><input id="phone" type="text" name="phone" value="<?php echo esc_attr(get_the_author_meta('phone', $user->ID)); ?>" class="regular-text"></td>
</tr>
<tr>
<th><lable for="team">Show on the team page</lable></th>
<td><input <?php if (get_the_author_meta('team',$user->ID)): ?>checked<?php endif; ?> id="team" type="checkbox" name="team" value="1"></td>
</tr>
</table>
<?php
}
}
add_action('show_user_profile', 'm_w_db_user_profile_show');
add_action('edit_user_profile', 'm_w_db_user_profile_show');
if (!function_exists('m_w_db_user_profile_update')) {
function m_w_db_user_profile_update($user_id) {
update_user_meta($user_id, 'job_title', sanitize_text_field($_POST['job_title']));
update_user_meta($user_id, 'phone', sanitize_text_field($_POST['phone']));
update_user_meta($user_id, 'team', $_POST['team'] ? $_POST['team'] : 0 );
}
}
add_action('personal_options_update', 'm_w_db_user_profile_update');
add_action('edit_user_profile_update', 'm_w_db_user_profile_update');
// Is this in the menu?
/**
* Check if post is in a menu
*
* @param $menu menu name, id, or slug
* @param $object_id int post object id of page
* @return bool true if object is in menu
*/
function cms_is_in_menu( $menu = null, $object_id = null ) {
// get menu object
$menu_object = wp_get_nav_menu_items( esc_attr( $menu ) );
// stop if there isn't a menu
if( ! $menu_object )
return false;
// get the object_id field out of the menu object
$menu_items = wp_list_pluck( $menu_object, 'object_id' );
// use the current post if object_id is not specified
if( !$object_id ) {
global $post;
$object_id = get_queried_object_id();
}
// test if the specified page is in the menu or not. return true or false.
return in_array( (int) $object_id, $menu_items );
}
// breadcrumbs function
function the_breadcrumb() {
global $post;
echo '<ul id="breadcrumbs">';
if (!is_home()) {
echo '<li><a href="';
echo get_option('home');
echo '">';
echo 'Homepage';
echo '</a></li><li class="separator">/</li>';
if (is_category()) {
echo '<li>';
the_category(' </li><li class="separator">/</li><li> ');
}
elseif (is_single() ) {
echo '<li><a href="'. get_permalink( get_option( 'page_for_posts' ) ).'" title="">Blog</a></li> <li class="separator">/</li>';
$single_title = single_post_title('', false);
echo '<li><strong>'. $single_title .' </strong></li>';
}
elseif (is_page()) {
if($post->post_parent){
$anc = get_post_ancestors( $post->ID );
$title = get_the_title();
foreach ( $anc as $ancestor ) {
$output = '<li><a href="'.get_permalink($ancestor).'" title="'.get_the_title($ancestor).'">'.get_the_title($ancestor).'</a></li> <li class="separator">/</li>';
}
echo $output;
echo '<strong title="'.$title.'"> '.$title.'</strong>';
} else {
echo '<li><strong> '.get_the_title().'</strong></li>';
}
}
elseif (is_tag()) {
echo '<li><a href="'. get_permalink( get_option( 'page_for_posts' ) ).'" title="">Blog</a></li> <li class="separator">/</li>';
$single_tag_title = single_tag_title('', false);
echo '<li><strong>'. $single_tag_title .' </strong></li>';
}
elseif (is_search()) {
echo"<li><strong>Search Results</strong>"; echo'</li>';
}
elseif (is_archive()) {
echo '<li><a href="'. get_permalink( get_option( 'page_for_posts' ) ).'" title="">Blog</a></li> <li class="separator">/</li>';
$single_tag_title = single_tag_title('', false);
{echo "<li><strong>Blog Archives</strong>"; echo'</li>';}
}
elseif (isset($_GET['paged']) && !empty($_GET['paged'])) {echo "<li>Blog Archives"; echo'</li>';}
}
elseif (is_tag()) {single_tag_title();}
elseif (is_home()) {
echo '<li><a href="';
echo get_option('home');
echo '">';
echo 'Homepage';
echo '</a></li><li class="separator">/</li>';
echo '<li><strong>Blog<strong></li>';
}
elseif (is_day()) {echo"<li>Archive for "; the_time('F jS, Y'); echo'</li>';}
elseif (is_month()) {echo"<li>Archive for "; the_time('F, Y'); echo'</li>';}
elseif (is_year()) {echo"<li>Archive for "; the_time('Y'); echo'</li>';}
elseif (is_author()) {echo"<li>Author Archive"; echo'</li>';}
elseif (isset($_GET['paged']) && !empty($_GET['paged'])) {echo "<li>Blog Archives"; echo'</li>';}
elseif (is_search()) {echo"<li>Search Results"; echo'</li>';}
echo '</ul>';
}<file_sep><?php
global $post;
$menuitems = wp_get_nav_menu_items( esc_attr( 'Primary Menu' ), array(
'post_parent' => 0,
'post_type' => 'nav_menu_item',
'post_status' => 'publish',
'posts_per_page' => -1
) );?>
<div class="main-items-holder">
<?php
foreach ($menuitems as $menuitem) {
$id = $menuitem->object_id;
$mypost = get_post($id);
setup_postdata($mypost);
?>
<!--[if IE 9]><div class="floatIE"><![endif]--><!--[if IE 8]><div class="floatIE"><![endif]-->
<div class="main-items-item">
<div class="main-items-item-img">
<div class="circle">
<img src="<?php echo types_render_field('icon', array('post_id' => $id,'url'=>'true')); ?>" alt="" style="display: block; border: 0">
</div>
<div class="gradient-line"></div>
</div>
<div class="main-items-item-copy">
<h3><?php echo get_the_title($id); ?></h3>
<p>
<?php the_excerpt(); ?>
<br>
<a href="<?php echo get_permalink($id); ?>">[Read more]</a>
</p>
</div>
</div>
<!--[if IE 9]></div><![endif]--><!--[if IE 8]></div><![endif]-->
<?php
}
?>
</div> | 31b04e8b838720ddfba91d2e5c0f049020f6ca7c | [
"JavaScript",
"PHP"
] | 13 | PHP | MartiRainstorm/MarshallWooldridgeWordpress | 7344b8573733a5d7179d19fa72fd57816a35b502 | 7fcb4a1aec06f4388b5c20de44deea2ea570194c |
refs/heads/master | <repo_name>CristianoPro/T2-Lista-de-elementos<file_sep>/Model/Circulo.java
package Model;
/**
*
* @author <NAME>
* tia 41612590
*
*/
public class Circulo {
private double raio;
private Ponto centro; // associacao Classe A possui B (=Ponto)
// construtor
public Circulo(double raio, Ponto centro) {
this.raio = raio;
this.centro = centro;
}
/*
* metodo decide se um ponto est� dentro do c�rculo.
*/
public boolean estaDentro(Ponto p) {
return centro.distancia(p) <= raio;
}
}
| 904d6db4d3c51eddb5f5cc2f4cced7430a11175d | [
"Java"
] | 1 | Java | CristianoPro/T2-Lista-de-elementos | a98275f589970a068aa78eee0b64f8d2f477e871 | 7981f1555a888710fe840aeb91df9182ddd2848d |
refs/heads/main | <repo_name>scratchytube/hey-frontend<file_sep>/src/MadLibs.js
import React, { useState } from 'react'
import MadLibsCompleted from './MadLibsCompleted'
const MadLibs = () => {
const [showForm, setShowForm] = useState(false)
const [noun, setNoun] = useState("")
const [weekendGoing, setWeekendGoing] = useState("")
const handleSubmit = e => {
e.preventDefault()
setShowForm(true)
}
return (
<div className="Madlib_form" >
<h2>Gimme some answers</h2>
<form>
<h3>What was you first AOL screenName?</h3>
<input
className="madlib_input"
type="text"
name="noun"
placeholder="noun plz"
value={noun}
onChange={(e) => setNoun(e.target.value)}
/>
<h3>How is your week going?</h3>
<input
className="madlib_inputer"
type="text"
name="word"
value={weekendGoing}
onChange={(e) => setWeekendGoing(e.target.value)}
/>
<br></br>
<br></br>
<button onClick={handleSubmit} >Submit Me!</button>
</form>
{showForm && <MadLibsCompleted noun={noun} week={weekendGoing} /> }
</div>
)
}
export default MadLibs<file_sep>/src/Pages/Home.js
import React from 'react'
const Home = () => {
return (
<div className="home" >
<h1>hey_</h1>
<p>We're just a little helper for your dating apps.</p>
<button>Get Started</button>
</div>
)
}
export default Home<file_sep>/src/GeneratorPictureForm.js
import React from 'react'
import './GeneratorPictureForm.css'
const GeneratorPictureForm = ({ profileData }) => {
console.log(profileData)
const misterImages = profileData.map((pictureData) => (
<img src={pictureData.picture.completed_pictures} alt="hehe's"/>
))
return (
<div className="picture__form" >
{ misterImages }
<input name="image" placeholder="upload your face" />
<button>Submit</button>
</div>
)
}
export default GeneratorPictureForm<file_sep>/src/FavPicsContainer.js
import React from 'react'
import FavPictureCards from './FavPictureCards'
import './FavPicsContainer.css'
const FavPicsContainer = ({profileData}) => {
const misterPictures = profileData.map((profilePicture) => (
<FavPictureCards pictureData={profilePicture.picture}/>
))
return (
<div className="fav__pics" >
{ misterPictures }
</div>
)
}
export default FavPicsContainer<file_sep>/src/MadLibsCompleted.js
import React from 'react'
const MadLibsCompleted = ({ noun, week }) => {
return (
<div className="completed_madlibs">
<p>{noun} was what they called me in high school</p>
<p>Yes my day is going {week}</p>
<p>Yes my week has been {week}</p>
<p>Yes my weekend should be {week}</p>
<button>Add to Favorites</button>
</div>
)
}
export default MadLibsCompleted | b3e8918a9f1f7e4bf9444b1d74f098a74b214efc | [
"JavaScript"
] | 5 | JavaScript | scratchytube/hey-frontend | b818c5643c6aba78b9e01f1e36d1c61d1c3efa61 | 7ae4f56783d1561e5347d1e648f24f756612a096 |
refs/heads/main | <repo_name>anhhuyvu/Software-Architecture-Principles<file_sep>/Software_architecture/LSP/fix.js
class Phone {
call() {
console.log("Calling....");
}
}
class Smartphone extends Phone {
taking_photo() {
console.log("Photo has been taken. Check your album");
}
}<file_sep>/Software_architecture/ISP/fix.js
class Military{
constructor(name){
this.name = name;
}
}
const mover = {
move(){
console.log(`${this.name} moving`);
}
}
const attacker = {
attack(enemy)
{
console.log(`${this.name} attacking ${enemy.name} for ${this.attackDamage} damage`);
}
}
const reporter = {
report(){
console.log(`${this.name} reported that ${this.signal}`);
}
}
class Warrior extends Military{
constructor(name,attackDamage,signal){
super(name);
this.attackDamage = attackDamage;
this.signal = signal;
}
}
Object.assign(Warrior.prototype,mover);
Object.assign(Warrior.prototype,attacker);
Object.assign(Warrior.prototype,reporter);
class Tank extends Military{
constructor(name,attackDamage)
{
super(name);
this.name = name;
this.attackDamage = attackDamage;
}
}
Object.assign(Tank.prototype,mover);
Object.assign(Tank.prototype,attacker);
class Barrier extends Military{
constructor(name){
super(name);
this.name = name;
}
}
const warrior = new Warrior("John",34,"Boom");
const tank = new Tank("Echo_2",45);
const barrier = new Barrier("Block A");
warrior.move();
warrior.attack(barrier);
warrior.report();
tank.move();
tank.attack(warrior);
<file_sep>/Software_architecture/ISP/violating.js
class Military {
constructor(name, attackDamage, signal) {
this.name = name;
this.attackDamage = attackDamage;
this.signal = signal;
}
move() {
console.log(`${this.name} moving...`);
}
attack(enemy) {
console.log(`${this.name} attacking ${enemy.name} for ${this.attackDamage} damage`);
}
report() {
console.log(`${this.name} reported that ${this.signal}`);
}
}
class Warrior extends Military { }
class Tank extends Military {
report() {
return null;
//Tank can't report
}
}
class Barrier extends Military {
report() {
return null;
//Barrier can't report.
}
move() {
return null;
//Barrier can't move.
}
}
const warrior = new Warrior("John", 34, "Yes Sir");
warrior.move();
warrior.report();
warrior.attack(Tank);<file_sep>/Software_architecture/SRP/violating.js
class Book {
constructor(title, author) {
this.title = title;
this.author = author;
}
getTitle() {
return this.title;
};
getAuthor() {
return this.author;
};
saveBook() {
console.log("Book has been saved");
};
}
// the book should only show the title and the author, it should not save the book.<file_sep>/Software_architecture/OCP/fix.js
class Laptop {
constructor(name) {
this.name = name;
}
getname(){}
}
class Asus extends Laptop {
getname() {
console.log("This is " + this.name);
}
}
class Dell extends Laptop {
getname() {
console.log("This is " + this.name);
}
}
class Macbook extends Laptop {
getname() {
console.log("This is " + this.name);
}
}
function printLaptop(laptops)
{
laptops.forEach(laptop =>{
console.log(laptop.name)
laptop.getname()
console.log(' ')
})
}
const laptops =[
new Asus("Asus"),
new Dell("Dell"),
new Macbook("Macbook")
]
printLaptop(laptops);<file_sep>/Software_architecture/LSP/violating.js
class Phone {
call(phone_number) {
console.log("Calling....");
}
taking_photo() {
console.log("photo has been taken. Check your album.");
}
}
let landLine = new Phone();
landLine.taking_photo();
// landLine can't take photo
| 91173b830028b6f193d7b5c3afe76589aa521bd0 | [
"JavaScript"
] | 6 | JavaScript | anhhuyvu/Software-Architecture-Principles | 8569648b95c846ab66ac0a671cf53ee3fbc180a3 | 359b440c563e270fe4192a7cc30e13303c3faff0 |
refs/heads/master | <repo_name>gusmanb/PlotManager<file_sep>/PlotManager/PlotProgrammer.cs
using System;
using System.Collections.Concurrent;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
namespace PlotManager
{
public static class PlotProgrammer
{
static double tempFactor = 249;
static readonly Dictionary<int, long> plotSizes = new Dictionary<int, long> {
{ 25, 600 * 1024 * 1024 },
{ 32, 102L * 1024L * 1024L * 1024L },
{ 33, 209L * 1024L * 1024L * 1024L },
{ 34, 430L * 1024L * 1024L * 1024L },
{ 35, 885L * 1024L * 1024L * 1024L }
};
static ConcurrentDictionary<Guid, Plotter> runningPlots = new ConcurrentDictionary<Guid, Plotter>();
static CancellationTokenSource cancelSrc;
public static string Log { get; private set; } = "";
public static event EventHandler LogUpdated;
public static event EventHandler NewPlot;
static int _KSize;
static int _BufferSize;
static int _ThreadCount;
static TemporalFolder[] _TempFolders;
static string[] _OutputFolders;
static int _MinInterval;
static int _MaxPhaseOne;
public static bool Running { get; set; }
public static bool Start(int KSize, int BufferSize, int ThreadCount, TemporalFolder[] TempFolders, string[] OutputFolders, int MinInterval, int MaxPhaseOne)
{
if (cancelSrc != null)
{
AppendLog("Programmer already running");
return false;
}
_KSize = KSize;
_BufferSize = BufferSize;
_ThreadCount = ThreadCount;
_TempFolders = TempFolders;
_OutputFolders = OutputFolders;
_MinInterval = MinInterval;
_MaxPhaseOne = MaxPhaseOne;
cancelSrc = new CancellationTokenSource();
ExecuteProgrammer();
AppendLog("Programmer has started");
Running = true;
return true;
}
public static bool Stop()
{
if (cancelSrc != null)
{
cancelSrc.Cancel();
return true;
}
return false;
}
public static bool PausePlotter(Guid id)
{
runningPlots.TryGetValue(id, out Plotter plot);
if (plot == null)
return false;
AppendLog("Pausing plot...");
plot.PausePlotter();
return true;
}
public static bool ResumePlotter(Guid id)
{
runningPlots.TryGetValue(id, out Plotter plot);
if (plot == null)
return false;
AppendLog("Resuming plot...");
plot.ResumePlotter();
return true;
}
public static void KillAllPlots()
{
AppendLog("Killing plots...");
foreach (var item in runningPlots.Values)
item.RunningPlot.Kill();
AppendLog("All plots are dead...");
}
static void ExecuteProgrammer()
{
Task.Run(async () =>
{
try
{
while (!cancelSrc.IsCancellationRequested)
{
if (_MinInterval != 0)
{
var latest = runningPlots.Values.OrderByDescending(p => p.RunningPlot.StartTime.Value).FirstOrDefault();
if (latest != null)
{
while ((DateTime.Now - latest.RunningPlot.StartTime.Value).TotalMinutes < _MinInterval)
{
AppendLog("Delaying plot start...");
await Task.Delay(5000, cancelSrc.Token);
cancelSrc.Token.ThrowIfCancellationRequested();
}
}
}
cancelSrc.Token.ThrowIfCancellationRequested();
int onPhase1 = 0;
do
{
onPhase1 = runningPlots.Values.Where(p => p.CurrentPhase == 1).Count();
if (onPhase1 >= _MaxPhaseOne)
{
AppendLog("Phase1 plot exceeded, delaying plot start...");
await Task.Delay(5000, cancelSrc.Token);
}
cancelSrc.Token.ThrowIfCancellationRequested();
} while (onPhase1 >= _MaxPhaseOne);
cancelSrc.Token.ThrowIfCancellationRequested();
TemporalFolder selectedTemp = null;
while (selectedTemp == null)
{
var grp = _TempFolders
.Select(f => new
{
Folder = f,
Running = runningPlots.Count(rp => rp.Value.TempFolder == f.Folder),
RunningPhaseOne = runningPlots.Count(rp => rp.Value.TempFolder == f.Folder && rp.Value.CurrentPhase == 1),
RunningUnderThree = runningPlots.Count(rp => rp.Value.TempFolder == f.Folder && rp.Value.CurrentPhase < 3),
FreeSpots = f.MaxConcurrency - runningPlots.Count(rp => rp.Value.TempFolder == f.Folder),
FreeSpace = GetFreeTemp(f.Folder)
})
.Where(g => g.FreeSpace >= plotSizes[_KSize] && g.FreeSpots > 0)
.OrderBy(g => g.Running)
.ThenBy(g => g.RunningPhaseOne)
.FirstOrDefault();
if (grp == null)
{
AppendLog("Temps too busy, delaying plot start...");
await Task.Delay(5000, cancelSrc.Token);
}
else
selectedTemp = grp.Folder;
cancelSrc.Token.ThrowIfCancellationRequested();
}
var selectedOutput = _OutputFolders
.Where(f => GetFreeDisc(f) >= plotSizes[_KSize])
.Select(f => new
{
Folder = f,
Running = runningPlots.Count(rp => rp.Value.OutputFolder == f)
})
.OrderBy(g => g.Running)
.FirstOrDefault();
if (selectedOutput == null)
{
AppendLog("Output destinations are full, stopping programmer...");
cancelSrc.Cancel();
}
cancelSrc.Token.ThrowIfCancellationRequested();
AppendLog($"Starting plot in '{selectedTemp.Folder}' to '{selectedOutput.Folder}'...");
Plotter pt = new Plotter(_KSize, _BufferSize, _ThreadCount, selectedTemp.Folder, selectedOutput.Folder);
pt.PlotFinished += Pt_PlotFinished;
if (NewPlot != null)
NewPlot(pt, EventArgs.Empty);
runningPlots[pt.PlotterId] = pt;
pt.StartPlotter();
}
}
catch { }
Running = false;
AppendLog("Programmer is stopped.");
try
{
var cancel = cancelSrc;
cancelSrc = null;
cancel.Dispose();
}
catch { }
});
}
private static long GetFreeTemp(string Path)
{
var runningInUnderThree = runningPlots.Where(p => p.Value.TempFolder == Path && p.Value.CurrentPhase < 3).Count();
var runningOverThree = runningPlots.Where(p => p.Value.TempFolder == Path && p.Value.CurrentPhase >= 3).Count();
double scaledUnderThree = (runningInUnderThree * tempFactor) / 100.0;
long toRemove = (long)(runningOverThree * plotSizes[_KSize] + (scaledUnderThree * plotSizes[_KSize]));
var info = new DriveInfo(Path.Substring(0, 1));
return Math.Max(0, info.AvailableFreeSpace - toRemove);
}
private static long GetFreeDisc(string Path)
{
var runningIn = runningPlots.Where(p => p.Value.OutputFolder == Path).Count();
long toRemove = runningIn * plotSizes[_KSize];
var info = new DriveInfo(Path.Substring(0, 1));
return Math.Max(0, info.AvailableFreeSpace - toRemove);
}
private static void Pt_PlotFinished(object sender, EventArgs e)
{
Plotter pt = sender as Plotter;
runningPlots.TryRemove(pt.PlotterId, out _);
}
private static void AppendLog(string newLog)
{
string[] lines = Log.Split("\r\n".ToCharArray(), StringSplitOptions.RemoveEmptyEntries);
List<string> finalLines = new List<string>();
int skip = Math.Max(0, lines.Length - 99);
finalLines.AddRange(lines.Skip(skip));
finalLines.Add(newLog);
Log = string.Join("\r\n", finalLines);
if (LogUpdated != null)
LogUpdated(null, EventArgs.Empty);
}
public static void KillPlots(Guid id)
{
runningPlots.TryGetValue(id, out Plotter plot);
if (plot == null)
{
AppendLog("Cannot find plot...");
return;
}
AppendLog("Killing plot...");
plot.RunningPlot.Kill();
AppendLog("Plot is dead...");
}
}
}
<file_sep>/PlotManager/Models/PlotterConfig.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace PlotManager
{
public class PlotterConfig
{
public int KSize { get; set; }
public int Buffer { get; set; }
public int Threads { get; set; }
public TemporalFolder[] TempFolders { get; set; }
public string[] OutputFolders { get; set; }
public int Interval { get; set; }
public int PlotsInPhaseOne { get; set; }
}
}
<file_sep>/PlotManager/TempSelector.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace PlotManager
{
public partial class TempSelector : Form
{
public string Folder { get; set; }
public int MaxConcurrency { get; set; }
public TempSelector()
{
InitializeComponent();
}
private void btnAccept_Click(object sender, EventArgs e)
{
if (string.IsNullOrWhiteSpace(txtFolder.Text))
{
MessageBox.Show("Select temporary folder.");
return;
}
Folder = txtFolder.Text;
MaxConcurrency = (int)nudConcurrency.Value;
DialogResult = DialogResult.OK;
Close();
}
private void btnSelect_Click(object sender, EventArgs e)
{
using (FolderBrowserDialog fb = new FolderBrowserDialog())
{
if (fb.ShowDialog() != DialogResult.OK)
return;
txtFolder.Text = fb.SelectedPath;
}
}
private void btnCancel_Click(object sender, EventArgs e)
{
DialogResult = DialogResult.Cancel;
Close();
}
}
}
<file_sep>/README.md
# PlotManager
Little chia plot manager
Get control over your plotting in order to maximize throughput.

<file_sep>/PlotManager/MainPlotter.cs
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace PlotManager
{
public partial class MainPlotter : Form
{
public MainPlotter()
{
InitializeComponent();
DoubleBuffered(lstRunningPlots, true);
PlotProgrammer.LogUpdated += PlotProgrammer_LogUpdated;
PlotProgrammer.NewPlot += PlotProgrammer_NewPlot;
}
private void PlotProgrammer_NewPlot(object sender, EventArgs e)
{
if (this.InvokeRequired)
this.BeginInvoke((MethodInvoker)(() => AddPlotter(sender as Plotter)));
else
AddPlotter(sender as Plotter);
}
void AddPlotter(Plotter plot)
{
plot.LogUpdated += Plot_LogUpdated;
plot.PhaseUpdated += Plot_PhaseUpdated;
plot.PlotFinished += Plot_PlotFinished;
ListViewItem lvi = new ListViewItem(new string[] {
DateTime.Now.ToString(),
plot.CurrentPhase.ToString(),
"",
plot.TempFolder,
plot.OutputFolder,
"Running"});
lvi.Tag = plot.PlotterId;
lstRunningPlots.BeginUpdate();
lstRunningPlots.Items.Add(lvi);
lstRunningPlots.EndUpdate();
}
private void Plot_PlotFinished(object sender, EventArgs e)
{
var plot = sender as Plotter;
plot.LogUpdated -= Plot_LogUpdated;
plot.PhaseUpdated -= Plot_PhaseUpdated;
plot.PlotFinished -= Plot_PlotFinished;
if (this.InvokeRequired)
this.BeginInvoke((MethodInvoker)(() => FinishPlot(sender as Plotter)));
else
FinishPlot(sender as Plotter);
}
void FinishPlot(Plotter plot)
{
lstRunningPlots.BeginUpdate();
var item = lstRunningPlots.Items.Cast<ListViewItem>().Where(lvi => lvi.Tag.ToString() == plot.PlotterId.ToString()).FirstOrDefault();
if (item != null)
item.SubItems[5].Text = "Finished";
lstRunningPlots.EndUpdate();
}
private void Plot_PhaseUpdated(object sender, EventArgs e)
{
if (this.InvokeRequired)
this.BeginInvoke((MethodInvoker)(() => UpdatePlotPhase(sender as Plotter)));
else
UpdatePlotPhase(sender as Plotter);
}
void UpdatePlotPhase(Plotter plot)
{
lstRunningPlots.BeginUpdate();
var item = lstRunningPlots.Items.Cast<ListViewItem>().Where(lvi => lvi.Tag.ToString() == plot.PlotterId.ToString()).FirstOrDefault();
if (item != null)
item.SubItems[1].Text = plot.CurrentPhase.ToString();
lstRunningPlots.EndUpdate();
}
private void Plot_LogUpdated(object sender, EventArgs e)
{
if (this.InvokeRequired)
this.BeginInvoke((MethodInvoker)(() => UpdatePlotLog(sender as Plotter)));
else
UpdatePlotLog(sender as Plotter);
}
void UpdatePlotLog(Plotter plot)
{
lstRunningPlots.BeginUpdate();
var item = lstRunningPlots.Items.Cast<ListViewItem>().Where(lvi => lvi.Tag.ToString() == plot.PlotterId.ToString()).FirstOrDefault();
if (item != null)
item.SubItems[2].Text = plot.LastLogEntry.ToString();
lstRunningPlots.EndUpdate();
}
private void PlotProgrammer_LogUpdated(object sender, EventArgs e)
{
if (this.InvokeRequired)
this.BeginInvoke((MethodInvoker)(() => UpdatePlotManagerLog()));
else
UpdatePlotManagerLog();
}
void UpdatePlotManagerLog()
{
txtLogProg.Text = PlotProgrammer.Log;
txtLogProg.SelectionStart = txtLogProg.Text.Length;
txtLogProg.SelectionLength = 0;
txtLogProg.ScrollToCaret();
if (!PlotProgrammer.Running)
{
button1.Enabled = true;
button2.Enabled = false;
}
}
private void button1_Click(object sender, EventArgs e)
{
if (lstTempFolders.Items.Count == 0 || lstOuts.Items.Count == 0)
{
MessageBox.Show("You must at least specify one temp folder and one output folder");
return;
}
if (!PlotProgrammer.Start((int)nudKSize.Value,
(int)nudBuffer.Value,
(int)nudThreads.Value,
lstTempFolders.Items.Cast<ListViewItem>().Select(lv => new TemporalFolder { Folder = lv.Text, MaxConcurrency = int.Parse(lv.SubItems[1].Text) }).ToArray(),
lstOuts.Items.Cast<string>().ToArray(),
(int)nudInterval.Value,
(int)nudPlotsPhase1.Value))
{
MessageBox.Show("Error starting plotter programmer!");
}
else
{
var fileName = Path.Combine(Environment.GetFolderPath(Environment.SpecialFolder.ApplicationData), "plotter.cfg");
File.WriteAllText(fileName, JsonConvert.SerializeObject(new PlotterConfig
{
KSize = (int)nudKSize.Value,
Buffer = (int)nudBuffer.Value,
Threads = (int)nudThreads.Value,
TempFolders = lstTempFolders.Items.Cast<ListViewItem>().Select(lv => new TemporalFolder { Folder = lv.Text, MaxConcurrency = int.Parse(lv.SubItems[1].Text) }).ToArray(),
OutputFolders = lstOuts.Items.Cast<string>().ToArray(),
Interval = (int)nudInterval.Value,
PlotsInPhaseOne = (int)nudPlotsPhase1.Value
}));
button1.Enabled = false;
button2.Enabled = true;
}
}
private void btnAddTemp_Click(object sender, EventArgs e)
{
using (TempSelector fb = new TempSelector())
{
if (fb.ShowDialog() != DialogResult.OK)
return;
lstTempFolders.Items.Add(new ListViewItem(new string[] { fb.Folder, fb.MaxConcurrency.ToString() }));
}
}
private void btnAddOutput_Click(object sender, EventArgs e)
{
using (FolderBrowserDialog fb = new FolderBrowserDialog())
{
if (fb.ShowDialog() != DialogResult.OK)
return;
lstOuts.Items.Add(fb.SelectedPath);
}
}
private void button2_Click(object sender, EventArgs e)
{
if (!PlotProgrammer.Stop())
MessageBox.Show("Error stopping the programmer!");
else
{
button1.Enabled = true;
button2.Enabled = false;
}
}
void DoubleBuffered(Control control, bool enable)
{
var doubleBufferPropertyInfo = control.GetType().GetProperty("DoubleBuffered", BindingFlags.Instance | BindingFlags.NonPublic);
doubleBufferPropertyInfo.SetValue(control, enable, null);
}
private void button3_Click(object sender, EventArgs e)
{
lstRunningPlots.BeginUpdate();
var items = lstRunningPlots.Items.Cast<ListViewItem>().Where(lvi => lvi.SubItems[5].Text == "Finished").ToArray();
if (items != null)
{
foreach (var item in items)
lstRunningPlots.Items.Remove(item);
}
lstRunningPlots.EndUpdate();
}
private void button4_Click(object sender, EventArgs e)
{
if (lstRunningPlots.SelectedItems.Count < 1)
{
MessageBox.Show("Select a plot to pause/resume");
return;
}
var item = lstRunningPlots.SelectedItems[0];
if (item.SubItems[5].Text == "Finished")
{
MessageBox.Show("Selected plot already finished");
return;
}
var id = (Guid)item.Tag;
if (item.SubItems[5].Text == "Paused")
{
if (PlotProgrammer.ResumePlotter(id))
item.SubItems[5].Text = "Running";
}
else
{
if (PlotProgrammer.PausePlotter(id))
item.SubItems[5].Text = "Paused";
}
}
private void button5_Click(object sender, EventArgs e)
{
if (MessageBox.Show("Warning!! This will kill all running plots leaving all the temp files in place, are you sure?", "Kill Plots", MessageBoxButtons.OKCancel) != DialogResult.OK)
return;
PlotProgrammer.KillAllPlots();
}
private void MainPlotter_FormClosing(object sender, FormClosingEventArgs e)
{
bool running = lstRunningPlots.Items.Cast<ListViewItem>().Where(lvi => lvi.SubItems[5].Text != "Finished").Any();
if (running)
{
e.Cancel = true;
MessageBox.Show("There are running plots, cancell all before closing PlotManager");
}
}
private void button6_Click(object sender, EventArgs e)
{
if (lstRunningPlots.SelectedItems.Count < 1)
{
MessageBox.Show("Select a plot to kill");
return;
}
var item = lstRunningPlots.SelectedItems[0];
if (item.SubItems[5].Text == "Finished")
{
MessageBox.Show("Selected plot already finished");
return;
}
if (MessageBox.Show("Warning!! This will kill the selected plot leaving all the temp files in place, are you sure?", "Kill Plot", MessageBoxButtons.OKCancel) != DialogResult.OK)
return;
var id = (Guid)item.Tag;
PlotProgrammer.KillPlots(id);
}
private void MainPlotter_Load(object sender, EventArgs e)
{
string configFile = Path.Combine(Application.StartupPath, "ChiaPath.txt");
if (!File.Exists(configFile))
{
MessageBox.Show("Cannot find chia path config file. Create a file in the application folder named \"ChiaPath.txt\" and place the full path to the chia executable.");
Task.Run(async () =>
{
await Task.Delay(1000);
Close();
});
return;
}
string path = File.ReadAllLines(configFile).FirstOrDefault();
if (string.IsNullOrWhiteSpace(path))
{
MessageBox.Show("Broken chia path config file. Create a file in the application folder named \"ChiaPath.txt\" and place the full path to the chia executable in its first line.");
Task.Run(async () =>
{
await Task.Delay(1000);
Close();
});
return;
}
if (!File.Exists(path))
{
MessageBox.Show($"Cannot find chia executable in \"{path}\"");
Task.Run(async () =>
{
await Task.Delay(1000);
Close();
});
return;
}
Plotter.ConfigureChiaPath(path);
}
private void bttnRemoveTemp_Click(object sender, EventArgs e)
{
if (lstTempFolders.SelectedItems != null && lstTempFolders.SelectedItems.Count > 0)
lstTempFolders.Items.Remove(lstTempFolders.SelectedItems[0]);
}
private void btnRemoveOutput_Click(object sender, EventArgs e)
{
if (lstOuts.SelectedIndex > -1)
lstOuts.Items.Remove(lstOuts.SelectedItem);
}
}
}
<file_sep>/PlotManager/Plotter.cs
using PlotManager.ConsoleAppManagement;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Text.RegularExpressions;
using System.Threading.Tasks;
namespace PlotManager
{
public class Plotter
{
static string chiaPath;
public static void ConfigureChiaPath(string Path)
{
chiaPath = Path;
}
ConsoleApp runningPlot;
public Guid PlotterId { get; private set; } = Guid.NewGuid();
public string Log { get; private set; } = "";
public int KSize { get; private set; }
public int BufferSize { get; private set; }
public int ThreadCount { get; private set; }
public string TempFolder { get; private set; }
public string OutputFolder { get; private set; }
public int CurrentPhase { get; private set; } = 1;
public bool Finished { get; private set; }
public string LastLogEntry { get; private set; } = "";
public ConsoleApp RunningPlot { get { return runningPlot; } }
Regex regPhase = new Regex("^Starting phase ([0-9])/4");
public event EventHandler PhaseUpdated;
public event EventHandler LogUpdated;
public event EventHandler PlotFinished;
public Plotter(int KSize, int BufferSize, int ThreadCount, string TempFolder, string OutputFolder)
{
this.KSize = KSize;
this.BufferSize = BufferSize;
this.ThreadCount = ThreadCount;
this.TempFolder = TempFolder;
this.OutputFolder = OutputFolder;
}
public void StartPlotter()
{
if (runningPlot == null)
{
runningPlot = new ConsoleApp(chiaPath, $"plots create -k {KSize} -b {BufferSize} -u 128 -r {ThreadCount} -t {TempFolder} -d {OutputFolder} -n 1 --override-k");
runningPlot.ConsoleOutput += RunningPlot_ConsoleOutput;
runningPlot.Exited += RunningPlot_Exited;
runningPlot.Run();
}
}
private void RunningPlot_Exited(object sender, EventArgs e)
{
Finished = true;
if (PlotFinished != null)
PlotFinished(this, EventArgs.Empty);
}
private void RunningPlot_ConsoleOutput(object sender, ConsoleOutputEventArgs e)
{
LastLogEntry = e.Line;
Log += "\r\n" + e.Line;
var match = regPhase.Match(e.Line);
if (match != null && match.Success)
{
CurrentPhase = int.Parse(match.Groups[1].Value);
if (PhaseUpdated != null)
PhaseUpdated(this, EventArgs.Empty);
}
if (LogUpdated != null)
LogUpdated(this, EventArgs.Empty);
}
public void PausePlotter()
{
if(runningPlot != null)
runningPlot.Pause();
}
public void ResumePlotter()
{
if (runningPlot != null)
runningPlot.Resume();
}
}
}
| 4c1870fca4186b34b8b5dfd9589e34611a1defef | [
"Markdown",
"C#"
] | 6 | C# | gusmanb/PlotManager | 3fb110757b39c23617639d0ef354ea744eb927e0 | e6f859b3d9d692b8794f8e577fd9fdbbc57ce139 |
refs/heads/master | <repo_name>stokic16/Exercise-202<file_sep>/README.md
# Exercise-202
<file_sep>/Exercise_202/src/exercise_202/SenderTableModel.java
package exercise_202;
import java.io.EOFException;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.util.ArrayList;
import javax.swing.table.AbstractTableModel;
/**
* @author <NAME>
*/
public class SenderTableModel extends AbstractTableModel{
private String[] names= {"Sender","Frequenz","Band"};
private ArrayList<Sender> senders = new ArrayList<>();
public void add(Sender s){
senders.add(s);
senders.sort(new CompareToFrequenz());
fireTableRowsInserted(senders.size()-1, senders.size()-1);
}
public void hideBand(){
names = new String[] {"Sender","Frequenz"};
fireTableStructureChanged();
}
public void showBand(){
names = new String[] {"Sender","Frequenz","Band"};
fireTableStructureChanged();
}
public void save(File f) throws Exception{
FileOutputStream fos = new FileOutputStream(f);
ObjectOutputStream oos = new ObjectOutputStream(fos);
for (Sender sender : senders) {
System.out.println("true");
oos.writeObject(sender);
}
oos.flush();
oos.close();
fos.flush();
fos.close();
}
public void load(File f)throws Exception{
FileInputStream fis = new FileInputStream(f);
ObjectInputStream ois = new ObjectInputStream(fis);
Object input=null;
try{
while((input=ois.readObject())!=null){
if(input instanceof Sender){
senders.add((Sender)input);
}
}
}catch(EOFException e){
e.printStackTrace();
}
fis.close();
ois.close();
}
@Override
public int getRowCount() {
return senders.size();
}
@Override
public int getColumnCount() {
return names.length;
}
@Override
public String getColumnName(int column) {
return names[column];
}
@Override
public Object getValueAt(int rowIndex, int columnIndex) {
return senders.get(rowIndex);
}
}
<file_sep>/Exercise_202/src/exercise_202/Sender.java
package exercise_202;
import java.io.Serializable;
/**
* @author <NAME>
*/
public class Sender implements Serializable{
private String name;
private double frequenz;
private Band band;
enum Band{
AM,FM;
}
public Sender(String name, double frequenz, Band band) {
this.name = name;
this.frequenz = frequenz;
this.band = band;
}
public Band getBand() {
return band;
}
public double getFrequenz() {
return frequenz;
}
public String getName() {
return name;
}
}
| 1bce64cb970b5c345f0da4872ca043b3ed80547c | [
"Markdown",
"Java"
] | 3 | Markdown | stokic16/Exercise-202 | 99d9378f534c1f7c6c8f55446934b3c59ac27c7a | 5efa72391095871825ccf4705fca82d4677545a9 |
refs/heads/master | <file_sep>html page for TriMet GIS Survey home page
<file_sep>google.charts.load('current', {'packages':['corechart']});
google.charts.setOnLoadCallback(drawStuff);
function drawStuff() {
var data = new google.visualization.DataTable();
data.addColumn('string', 'Year');
data.addColumn('number', 'Cost');
data.addColumn({type: 'string', role: 'annotation'});
data.addRows([
["2012", 71, '$71'],
["2016", 48, '$48']
]);
var formatter = new google.visualization.NumberFormat(
{ prefix: '$'}
);
formatter.format(data, 1);
/*var view = new google.visualization.DataView(data);
view.setColumns([0, 1,
{ calc: "stringify",
sourceColumn: 1,
type: "string",
role: "annotation" },
2]);*/
var view = new google.visualization.DataView(data);
view.setColumns([0, 1, 2]);
var options = {
title: 'Cost Per Sample Hour',
titleTextStyle: {
fontName: 'Arial',
fontSize: 14,
bold: true
},
width: 500,
legend: { position: 'none' },
chart: { title: 'Cost Per Sample Hour Comparison' },
bar: { groupWidth: "70%" },
vAxis: {format: 'currency'},
colors:['#0069aa']
};
var chart = new google.visualization.ColumnChart(document.getElementById('cost_div'));
chart.draw(view, options);
}; | 01e6a44380d8ef410225b7c5af46c25a4455062a | [
"Markdown",
"JavaScript"
] | 2 | Markdown | gis-survey/gissurvey-homepage | bdfaace3b87179058f7a237368e48e9e6bab4286 | ec541fe317380a35db57b19f4fd7fe8c6bc4f18d |
refs/heads/master | <repo_name>eyesicedover/AnimalSanctuary<file_sep>/AnimalSanctuaryTests/ControllerTests/VetControllerTest.cs
using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using AnimalSanctuary.Controllers;
using AnimalSanctuary.Models.Repositories;
using AnimalSanctuary.Models;
using Microsoft.AspNetCore.Mvc;
using Moq;
using System.Linq;
namespace AnimalSanctuaryTests.ControllerTests
{
[TestClass]
class VetControllerTest
{
Mock<IVetRepository> mock = new Mock<IVetRepository>();
private void DbSetup()
{
mock.Setup(m => m.Vets).Returns(new Vet[]
{
new Vet {VetId = 1, Name = "<NAME>", Specialty = "Horsebonologist"},
new Vet {VetId = 1, Name = "<NAME>", Specialty = "Holder of Eldritch Frog Knowledge"}
}.AsQueryable());
}
[TestMethod]
public void Mock_VetIndexContainsModelData_List()
{
//Arrange
DbSetup();
ViewResult indexView = new VetController(mock.Object).Index() as ViewResult;
//Act
var result = indexView.ViewData.Model;
//Assert
Assert.IsInstanceOfType(result, typeof(List<Vet>));
}
}
}
| 3493e34548a58451d3a5c974ad2208f5b895beab | [
"C#"
] | 1 | C# | eyesicedover/AnimalSanctuary | cc90add7b015a7f3350ec305e38a11d0a4987b16 | 9338ac8e9bb9ca618dd41cd6ab96504ff9abb13a |
refs/heads/master | <repo_name>NinjaTrappeur/blog-1<file_sep>/README.md
Blog
====
> My personal blog.
Description
-----------
This repository contains the scripts to build my static blog, together
with the articles Markdown source.
Everything is built around a [makefile](Makefile). Markdown parsing is
done with the [markdown-it] library, [Jade] is used for pages
templating, [Stylus] for CSS preprocessing, and the awesome [Babel] to
transpile ES6 to ES5.
[markdown-it]: https://github.com/markdown-it/markdown-it
[Jade]: http://jade-lang.com/
[Stylus]: http://learnboost.github.io/stylus/
[Babel]: https://babeljs.io/
Everything in this repository is in the public domain, thanks to the
[Unlicense](http://unlicense.org/), but if you want to hack on this
project, you probably want to remove the content and keep only the
engine; just remove everything in the `public` directory except the
`*.list` files (used for index, posts list and RSS feed).
I may separate the engine from the content if there is some need to
reuse the engine elsewhere, to make it more maintainable.
Installation
------------
You need a Node.js compliant environment, and GNU `make` to build.
```sh
npm install
make
```
Usage
-----
### Update everything
```sh
make
```
Only the stuff that changed since last compilation will be updated.
### Create a new post
```sh
make new
```
This will drop you in your editor, in a new prepared draft, with a title
and the current date. When exiting, the post will be moved to a dated
directory, with a slug derived from the page title.
Make sure to update the site after!
### Create a new page
Just create a Markdown file anywhere in the `public` directory, and it
will be compiled to HTML upon next build. You probably want to link to
this page from another page/post, otherwise it won't be discoverable.
<file_sep>/Makefile
PATH := bin:dist:node_modules/.bin:$(PATH)
MD = $(shell find public -type f -name '*.md') public/index.md public/posts.md
MD_HTML = $(MD:.md=.html)
POSTS = $(shell find public -mindepth 2 -type f -name '*.md' | egrep '^public/[0-9]{4}/')
POSTS_HTML = $(POSTS:.md=.html)
JS = $(shell find src -type f -not -name '.*')
JS_DIST = $(addprefix dist/,$(notdir $(JS)))
STYLUS = $(shell find stylus -type f -name '*.styl')
VIEWS = $(shell find views -type f -name '*.jade')
LIST = (echo; cat; echo) | between-tags '<!-- BEGIN LIST -->' '<!-- END LIST -->'
.SILENT:
all: $(JS_DIST) public/css/main.css $(MD_HTML) public/feed.xml
public/css/main.css: \
node_modules/normalize.css/normalize.css \
node_modules/highlight.js/styles/zenburn.css \
stylus/main.css
@echo generate $@
cleancss $^ > $@
stylus/main.css: $(STYLUS)
echo stylus stylus/main.styl
stylus -u autoprefixer-stylus stylus/main.styl
public/index.md: public/index.md.list bin/list $(POSTS_HTML)
echo update $@
list index $(POSTS_HTML) | $(LIST) $< > $@
public/posts.md: public/posts.md.list bin/list $(POSTS_HTML)
echo update $@
list posts $(POSTS_HTML) | $(LIST) $< > $@
public/feed.xml: public/feed.xml.list bin/list $(POSTS_HTML)
echo update $@
list feed $(POSTS_HTML) | $(LIST) $< > $@
dist/%.js: src/%.js
echo babel $<
babel < $< > $@
dist/%: src/%
echo babel $<
babel < $< > $@
chmod +x $@
%.html: %.part.html dist/render $(VIEWS)
echo render $<
render $< | html-minifier -c .html-minifier > $@
.PRECIOUS: %.part.html
%.part.html: %.md dist/md
echo 'md $<'
md < $< > $@
new:
new
<file_sep>/bin/meta
#!/bin/sh -e
#
# Get title (not Markdown parsed) and date from input Markdown file.
#
head=$(head -3 | sed 's/^# //;/^=*$/d')
title=$(echo "$head" | head -1)
date=$(echo "$head" | tail -1)
time=$(moment "$date" x)
echo "$title"
echo "$date"
echo "$time"
<file_sep>/src/render
#!/usr/bin/env node
//
// Render given partial HTML file with layout.
//
// * Page title can be set in a `<title>` tag in the first line.
// * Layout name is given as first argument.
//
import concat from 'stream-concat-promise'
import fs from 'fs'
import jade from 'jade'
import * as util from './util'
const file = process.argv[2]
const base = util.base(file)
const layout = util.layout(file)
const render = locals =>
jade.renderFile(__dirname + `/../views/${layout}.jade`, locals)
const findTitle = html => {
const match = html.match(/^<title>(.*)<\/title>/)
return !match
? ['', html]
: [match[1], html.substr(match[0].length)]
}
concat(fs.createReadStream(file), { encoding: 'string' })
.then(findTitle)
.then(([title, html]) => render({ file, base, layout, title, html }))
.then(console.log)
.then(null, require('promise-done'))
<file_sep>/src/md
#!/usr/bin/env node
//
// Render input Markdown file as HTML.
//
import concat from 'stream-concat-promise'
import mdit from 'markdown-it'
const md = mdit({
html: true,
typographer: true
})
md.use(require('markdown-it-anchor'), {
level: 2, // Do not permalink `<h1>`.
permalink: true,
parmalinkBefore: true
})
md.use(require('markdown-it-deflist'))
md.use(require('markdown-it-footnote'))
md.use(require('markdown-it-highlightjs'), { auto: false })
md.use(require('markdown-it-title'))
const env = {}
concat(process.stdin, { encoding: 'string' })
.then(src => md.render(src, env))
.then(html => console.log(`<title>${env.title}</title>\n${html}`))
.then(null, require('promise-done'))
<file_sep>/public/2014/07/hello-from-the-future.md
Hello from the future
=====================
July 01, 2014
This post is a virtual first post, to indicate that this blog really
began in March 2015, with [this post](../../2015/03/coming-soon.html).
Everything published before is articles I planned to write, but didn't
wrote because I didn't have a blog yet. Once my blog opened, I started
to publish back this stuff in the past, because the original date was
more relevant, than the actual publication date.
<file_sep>/bin/list
#!/bin/sh -e
#
# Create the list of posts for different contexts.
#
path() {
sed 's,public/,,'
}
xml() {
read _ post _ date
path=$(echo "$post" | path)
part=$(echo "$post" | sed 's/\.html$/.part.html/')
title=$(head -1 "$part" | sed 's,<title>,,;s,</title>,,')
url="https://www.codejam.info/$path"
cat << EOF
<item>
<title>$title</title>
<link>$url</link>
<guid>$url</guid>
<pubDate>$date</pubDate>
<description><![CDATA[
$(sed '1,3d;s,<a class="header-anchor" href="[^"]*">¶</a>,,' "$part")
]]></description>
</item>
EOF
}
md() {
read _ post title date
path=$(echo "$post" | path)
echo "* [$title]($path) <small>$date</small>"
}
meta_loop() {
for post in "$@"; do
md=$(echo "$post" | sed 's/\.html$/\.md/')
meta < "$md" | {
read title
read date
read time
echo "$time~$post~$title~$date"
}
done
}
loop() {
while read line; do
echo "$line" | IFS='~' "$1"
done
}
index() {
meta_loop "$@" | sort -r | head -15 | loop md
}
posts() {
meta_loop "$@" | sort -r | loop md
}
feed() {
meta_loop "$@" | sort -r | head -30 | loop xml
}
action=$1; shift
case "$action" in
index) index "$@" ;;
posts) posts "$@" ;;
feed) feed "$@" ;;
*) echo "Unknown action: $action" >&2; exit 1 ;;
esac
<file_sep>/bin/new
#!/bin/sh -e
#
# Create a new post.
#
# * Open editor on a draft file.
# * Extract the title slug and move the post in a dated directory.
#
if [ ! -f draft.md ]; then
printf 'Title\n=====\n%s\n\n\n' "$(date '+%B %d, %Y')" > draft.md
fi
$EDITOR draft.md
bin/meta < draft.md | {
read title
read date
slug=$(slugify "$(clean "$title")")
date=$(moment "$date" YYYY/MM)
mkdir -p "public/$date"
mv -i draft.md "public/$date/$slug.md"
echo "public/$date/$slug.md"
}
<file_sep>/public/network.md
Network
=======
IRC
---
Type | URL
------------------|-----
Default | [`irc://irc.codejam.info:6667/#general`](irc://irc.codejam.info:6667/#general)
SSL (recommended) | [`ircs://irc.codejam.info:6697/#general`](ircs://irc.codejam.info:6697/#general)
Web client | [`https://irc.codejam.info/?channels=general`](https://irc.codejam.info/?channels=general)
Git repositories
----------------
* [GitHub](https://github.com/valeriangalliat?tab=repositories)
* [Gogs](https://git.codejam.info/val) (mirror)
Misc
----
* [IP echo service](http://ip.codejam.info/) ([source](https://github.com/valeriangalliat/cgip))
<file_sep>/src/moment
#!/usr/bin/env node
//
// Display date with format.
//
import moment from 'moment'
console.log(moment(Date.parse(process.argv[2])).format(process.argv[3]))
<file_sep>/public/val.md
<NAME>
================
* Joined `#humanity` on July 20, 1995
* Spawned in Grenoble, France
* Currently in Montreal, Quebec
Contact
-------
Email/<abbr title="XMPP/Jabber">JID</abbr>
: `val`, at this very domain
GPG
: [`F489B457`](https://val.codejam.info/public/public.key.txt)
<small>(<code>DF3E D5DE 9E05 BF01 FAA7 8B37 CE76 5B32 F489 B457</code>)</small>
IRC
: * `val` on [`irc.codejam.info`](network.html#irc)
* `val` on [`ssl.neutralnetwork.org`](https://ssl.neutralnetwork.org/)
* `valerian` on [freenode](https://freenode.net/)
About
-----
* [Blog](.)
* [Twitter](https://twitter.com/valeriangalliat)
* [GitHub](https://github.com/valeriangalliat)
* [Gogs](https://git.codejam.info/val)
* [Resume](https://val.codejam.info/public/cv.en.pdf)
* [Sonerezh](https://www.sonerezh.bzh/) <small>([coming soon] -- beware, I have a
badass funk playlist)</small>
[coming soon]: 2015/03/coming-soon.html "The coming soon trap"
| 2bb34e97637a6fb23b665a4952d8dc04a562e1c9 | [
"Markdown",
"JavaScript",
"Makefile",
"Shell"
] | 11 | Markdown | NinjaTrappeur/blog-1 | f6d1fa41e753abc014bff066a1c9e61cf24fbb8c | 9f1ea322634f55cb3f2f92a6b50e945c78e3b842 |
refs/heads/master | <repo_name>bhatiyaarp/restapi_django<file_sep>/rest/api/face/retinaface/recog_support.py
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
from keras.layers import Lambda, Input
from keras.models import Model
from keras import backend as K
from .rface_model.lresnet100e_ir import LResNet100E_IR
from .utils.umeyama import umeyama
import tensorflow as tf
from tensorflow.python.platform import gfile
import numpy as np
import sys
import os
from .face_detection import detect_and_align
from . import id_data
from scipy import misc
import re
import cv2
import time
import pickle
from scipy import misc
import json
import glob
def load_data(image_paths, do_random_crop, do_random_flip, image_size, do_prewhiten=True):
nrof_samples = len(image_paths)
images = np.zeros((nrof_samples, image_size, image_size, 3))
for i in range(nrof_samples):
img = misc.imread(image_paths[i])
if img.ndim == 2:
img = to_rgb(img)
if do_prewhiten:
img = prewhiten(img)
img = crop(img, do_random_crop, image_size)
img = flip(img, do_random_flip)
images[i, :, :, :] = img
return images
def find_matching_id(id_dataset, embedding):
threshold = 0.65
min_dist = 10.0
matching_id = None
for id_data in id_dataset:
dist = get_embedding_distance(id_data.embedding, embedding)
if dist < threshold and dist < min_dist:
min_dist = dist
matching_id = id_data.name
return matching_id, min_dist
def get_embedding(sess, imgfile):
images_placeholder = tf.get_default_graph().get_tensor_by_name("input:0")
embeddings = tf.get_default_graph().get_tensor_by_name("embeddings:0")
phase_train_placeholder = tf.get_default_graph().get_tensor_by_name("phase_train:0")
embedding_size = 128
image_size = 160
emb_array = np.zeros((1, embedding_size))
# cropped = flip(imgfile, False)
# scaled= misc.imresize(cropped, (image_size, image_size), interp='bilinear')
# scaled = cv2.resize(cropped, (input_image_size,input_image_size),interpolation=cv2.INTER_CUBIC)
images = np.zeros((1, image_size, image_size, 3))
img = prewhiten(imgfile)
images[0, :, :, :] = img
images = prewhiten(images)
feed_dict = {images_placeholder: images, phase_train_placeholder: False}
emb_array = sess.run(embeddings, feed_dict=feed_dict)
return emb_array
def resize_tensor(size):
'''
load_rface_model() supporting function
size: input image size of model
'''
input_tensor = Input((None, None, 3))
output_tensor = Lambda(lambda x: tf.image.resize_bilinear(x, [size, size]))(input_tensor)
return Model(input_tensor, output_tensor)
def l2_norm(latent_dim):
'''
load_rface_model() supporting function
size: input image size of model
'''
input_tensor = Input((latent_dim,))
output_tensor = Lambda(lambda x: K.l2_normalize(x))(input_tensor)
return Model(input_tensor, output_tensor)
def align_face(im, src, size):
'''
load_rface_model() supporting function
size: input image size of model
'''
dst = np.array([
[30.2946, 51.6963],
[65.5318, 51.5014],
[48.0252, 71.7366],
[33.5493, 92.3655],
[62.7299, 92.2041]], dtype=np.float32)
dst[:, 0] += 8.0
dst = dst / 112 * size
M = umeyama(src, dst, True)[0:2]
warped = cv2.warpAffine(im, M, (size, size), borderValue=0.0)
return warped
def load_rface_model():
classes = 512
latent_dim = classes
input_resolution = 112
weights_path = "main/model/resource/face_recog/rface_model/lresnet100e_ir_keras.h5"
lresnet100e_ir = LResNet100E_IR(weights_path=weights_path)
input_tensor = Input((None, None, 3))
resize_layer = resize_tensor(size=input_resolution)
l2_normalize = l2_norm(latent_dim)
output_tensor = l2_normalize(lresnet100e_ir(resize_layer(input_tensor)))
net = Model(input_tensor, output_tensor)
net.trainable = False
print("Network Built...")
return net
def get_aligned_face(pnet, rnet, onet, imagedata, detect_multiple_faces, margin, input_resolution):
landmarks = None
try:
num_faces, landmarks, bboxes, face_detection_score, cropped_faces = detect_and_align.get_face(
None, pnet, rnet, onet, imagedata, detect_multiple_faces, margin)
except Exception as e:
print(e)
num_faces = 0
# num_faces = len(bboxes)
if num_faces == 0:
return num_faces, None
elif num_faces == 1:
landmarks = np.asarray(landmarks, dtype='float32')
points = landmarks.reshape((2, 5)).T
landmarks = np.zeros((5, 2), dtype=float)
landmarks[:, 0] = points[:, 1]
landmarks[:, 1] = points[:, 0]
landmarks = np.asarray([landmarks])
# print(landmarks)
# print(bboxes)
#################
if margin > 0:
## Left Eye
landmarks[0][0][0] = np.maximum(landmarks[0][0][0] - margin / 2, 0) # Y Component
landmarks[0][0][1] = np.maximum(landmarks[0][0][1] - margin / 2, 0) # X Component
## Right Eye
landmarks[0][1][0] = np.maximum(landmarks[0][1][0] - margin / 2, 0) # Y Component
landmarks[0][1][1] = np.minimum(landmarks[0][1][1] + margin / 2, imagedata.shape[1]) # X Component
## Left Mouth
landmarks[0][3][0] = np.minimum(landmarks[0][3][0] + margin / 2, imagedata.shape[1]) # Y Component
landmarks[0][3][1] = np.maximum(landmarks[0][3][1] - margin / 2, 0) # X Component
## right Mouth
landmarks[0][4][0] = np.minimum(landmarks[0][4][0] + margin / 2, imagedata.shape[1]) # Y Component
landmarks[0][4][1] = np.minimum(landmarks[0][4][1] + margin / 2, imagedata.shape[1]) # X Component
####################
aligned_face = align_face(imagedata, landmarks[0][..., ::-1], input_resolution)
return num_faces, aligned_face
elif num_faces > 1:
if detect_multiple_faces == 0:
return num_faces, None
else:
landmarks = np.asarray(landmarks[:10], dtype='float32')
points = landmarks.reshape((2, 5)).T
landmarks = np.zeros((5, 2), dtype=float)
landmarks[:, 0] = points[:, 1]
landmarks[:, 1] = points[:, 0]
landmarks = np.asarray([landmarks])
# print(landmarks)
# print(bboxes)
#################
if margin > 0:
## Left Eye
landmarks[0][0][0] = np.maximum(landmarks[0][0][0] - margin / 2, 0) # Y Component
landmarks[0][0][1] = np.maximum(landmarks[0][0][1] - margin / 2, 0) # X Component
## Right Eye
landmarks[0][1][0] = np.maximum(landmarks[0][1][0] - margin / 2, 0) # Y Component
landmarks[0][1][1] = np.minimum(landmarks[0][1][1] + margin / 2, imagedata.shape[1]) # X Component
## Left Mouth
landmarks[0][3][0] = np.minimum(landmarks[0][3][0] + margin / 2, imagedata.shape[1]) # Y Component
landmarks[0][3][1] = np.maximum(landmarks[0][3][1] - margin / 2, 0) # X Component
## right Mouth
landmarks[0][4][0] = np.minimum(landmarks[0][4][0] + margin / 2, imagedata.shape[1]) # Y Component
landmarks[0][4][1] = np.minimum(landmarks[0][4][1] + margin / 2, imagedata.shape[1]) # X Component
####################
aligned_face = align_face(imagedata, landmarks[0][..., ::-1], input_resolution)
return num_faces, aligned_face
def get_embedding_rface(net, imgfile):
'''
imgfile: image numpy narray
'''
input_array = imgfile[np.newaxis, ...]
# print(input_array.shape)
embedding = np.asarray(net.predict([input_array]), dtype='float32') # embedding = [[feature_vectors]]
return embedding[0]
def get_face_id_new(emb_array, model, class_names):
predictions = model.predict_proba(emb_array)
# print(predictions)
best_class_indices = np.argmax(predictions, axis=1)
# print(best_class_indices)
best_class_probabilities = predictions[np.arange(len(best_class_indices)), best_class_indices]
best_class_probability = best_class_probabilities[0]
best_class_indice = best_class_indices[0]
# print(best_class_probabilities)
print("Best class Detected, Probobility", class_names[best_class_indice], best_class_probability)
matching_id = class_names[best_class_indice]
'''
if best_class_probability > 0.35:
matching_id = class_names[best_class_indice]
else:
matching_id = 'Unknown'
'''
return matching_id
def verify_same_class_photos(tempenrollmentfolder_json, threshold=0.80):
# threshold = 1.0
testfolder = tempenrollmentfolder_json
file_names = os.listdir(testfolder)
num_images_test = len(file_names)
print(tempenrollmentfolder_json, num_images_test)
dist = []
for i in range(num_images_test - 1):
filepath1 = os.path.join(testfolder, file_names[i])
with open(filepath1, 'r') as f1:
result1 = json.load(f1)
edata1 = result1['data']
for j in range(i + 1, num_images_test, 1):
filepath2 = os.path.join(testfolder, file_names[j])
with open(filepath2, 'r') as f2:
result2 = json.load(f2)
edata2 = result2['data']
dist.append(get_embedding_distance(edata1, edata2))
max_dist = max(np.array(dist))
if max_dist < threshold:
verification = 1
else:
verification = 0
return verification, dist
def verification_user(emb_array, enrollfolder_JSON_path, threshold):
'''
loads emebeddings from ./enrollment_data_json/class_id/ directory and
then calculates distance.
enrollfolder_JSON_path: full path to class id whose embeddings are to be matched with emb_array.
'''
# 0.6
count = 0
dist_all = []
for filename in os.listdir(enrollfolder_JSON_path):
filepath = os.path.join(enrollfolder_JSON_path, filename)
print(filepath)
with open(filepath, 'r') as f1:
result = json.load(f1)
edata = result['data']
dist = get_embedding_distance(edata, emb_array)
dist_all.append(dist)
if dist < threshold:
count += 1
if count > 0: # 1 # check if >0 images match then verification status will be 1
verification = 1
else:
verification = 0
return verification, dist_all
# Modified by <NAME> on 18-10-2020
def verification_user_DB(emb_array, db_embeddings, threshold):
'''
takes list of embeddings of a perticular user and emb_aaray embed,
then calculates distance of emb_array with list of embeds to check if uploaded
images are of same userid which is provided while enrolling.
'''
count = 0
dist_all = []
for edata in db_embeddings:
dist = get_embedding_distance(edata, emb_array)
dist_all.append(dist)
if dist < threshold:
count += 1
if count > 0: # 1 # check if >0 images match then verification status will be 1
verification = 1
else:
verification = 0
return verification, dist_all
def verification_user_from_memoryEmbeddings(emb_array, cls_embeddings):
'''
find distance of passed emb_array with memory loaded embeddings (dictionary of embeddings)
emb_array: image features
cls_embeddings: list of embeddings for user to be verified
return: verification status and dist of emb_array with matching embedding in list.
'''
threshold = 0.90 # 0.6
count = 0
dist_all = []
for embeds in cls_embeddings:
edata = embeds
dist = get_embedding_distance(edata, emb_array)
dist_all.append(dist)
if dist < threshold:
count += 1
if count > 0: # 1 # check if >0 images match then verification status will be 1
verification = 1
else:
verification = 0
return verification, dist_all
def verify_id(emb_array, enrollfolder_JSON_path, threshold=0.66):
# threshold = 0.66 #0.73
# testfolder = "main/data/enrollment_data_json/"
testfolder = enrollfolder_JSON_path
folders = os.listdir(testfolder)
dist = []
classes = []
for fold in folders:
file_names = os.listdir(os.path.join(testfolder, fold))
num_images_test = min(8, len(file_names))
for i in range(num_images_test):
filepath = os.path.join(testfolder, fold, file_names[i])
with open(filepath, 'r') as f1:
try:
result = json.load(f1)
except Exception as e:
print(e)
print(filepath)
edata = result['data']
dist.append(get_embedding_distance(edata, emb_array))
classes.append(fold)
# dist = np.array(dist)
min_dist = min(np.array(dist))
min_index = dist.index(min_dist)
class_id = classes[min_index]
verification = 0
if min_dist <= threshold:
verification = 1
else:
verification = 0
return verification, min(dist), class_id
def verify_id_db(emb_array, embeddings_dict, threshold=0.66):
# threshold = 0.66 #0.73
min_dist = 0
matched_class_id = ''
verification_result = 0
enrolled_users = embeddings_dict.keys()
for key in enrolled_users:
user_embeds_list = embeddings_dict[key]
for emb in user_embeds_list:
dist = get_embedding_distance(emb, emb_array)
if dist<threshold:
min_dist=dist
matched_class_id=key
verification_result = 1
return verification_result, min_dist, matched_class_id
def verify_id_json(emb_array, embeddings):
'''
author - Ajay
compares given embeddings with embeddings dictionay
emb_array: Embedding vector of an image
embeddings: pre-loaded embeddings dictionary of all users
return : verification status, min distance, class id matched with
'''
threshold = 0.75 # 0.55
dist = []
classes = []
for fold in embeddings.keys():
embeds_list = embeddings[fold]
nrof_images = len(embeds_list)
for i in range(nrof_images):
edata = embeds_list[i]
dist.append(get_embedding_distance(edata, emb_array))
classes.append(fold)
# print(dist)
# dist = np.array(dist)
min_dist = min(np.array(dist))
min_index = dist.index(min_dist)
class_id = classes[min_index]
print("Minimum dist with class: ", class_id)
if min_dist < threshold:
verification = 1
else:
verification = 0
return verification, min(dist), class_id
def identify_verify_id_new(emb_array, userid):
'''
used for BSID
'''
threshold = 0.73
testfolder = "main/data/enrollment_data_json/" + str(userid)
file_names = os.listdir(testfolder)
# file_paths = [os.path.join(testfolder, img) for img in image_names]
num_images_test = min(16, len(file_names))
dist = []
for i in range(num_images_test):
filepath = os.path.join(testfolder, file_names[i])
with open(filepath, 'r') as f1:
result = json.load(f1)
edata = result['data']
dist.append(get_embedding_distance(edata, emb_array))
# print(dist)
if (min(dist)) < threshold:
verification = 1
else:
verification = 0
return verification, min(dist)
def get_face_id(imgfile):
classifier_filename = "main/model/resource/classifier/SVMclassifier.pkl"
with open(classifier_filename, 'rb') as infile:
(model, class_names) = pickle.load(infile)
with tf.Graph().as_default():
gpu_options = tf.GPUOptions(per_process_gpu_memory_fraction=0.5)
sess = tf.Session(config=tf.ConfigProto(gpu_options=gpu_options, log_device_placement=False))
with sess.as_default():
modeldir = "main/model/resource/face_recog/pre_model/20170511-185253.pb"
load_model(modeldir)
images_placeholder = tf.get_default_graph().get_tensor_by_name("input:0")
embeddings = tf.get_default_graph().get_tensor_by_name("embeddings:0")
phase_train_placeholder = tf.get_default_graph().get_tensor_by_name("phase_train:0")
embedding_size = embeddings.get_shape()[1]
image_size = 160
emb_array = np.zeros((1, embedding_size))
# cropped = flip(imgfile, False)
# scaled= misc.imresize(cropped, (image_size, image_size), interp='bilinear')
# scaled = cv2.resize(cropped, (input_image_size,input_image_size),interpolation=cv2.INTER_CUBIC)
images = np.zeros((1, image_size, image_size, 3))
img = prewhiten(imgfile)
images[0, :, :, :] = img
images = prewhiten(images)
feed_dict = {images_placeholder: images, phase_train_placeholder: False}
emb_array = sess.run(embeddings, feed_dict=feed_dict)
predictions = model.predict_proba(emb_array)
# print(predictions)
best_class_indices = np.argmax(predictions, axis=1)
# print(best_class_indices)
best_class_probabilities = predictions[np.arange(len(best_class_indices)), best_class_indices]
best_class_probability = best_class_probabilities[0]
best_class_indice = best_class_indices[0]
# print(best_class_probabilities)
print(class_names[best_class_indice], best_class_probability)
matching_id = class_names[best_class_indice]
'''
if best_class_probability > 0.35:
matching_id = class_names[best_class_indice]
else:
matching_id = 'Unknown'
'''
return matching_id
def identify_verify_id(imagefile, userid):
'''
for BSID
'''
threshold = 0.80
print(userid)
testfolder = "main/data/enrollment_data/" + str(userid)
image_names = os.listdir(testfolder)
image_paths = [os.path.join(testfolder, img) for img in image_names]
nrof_images = len(image_names)
image_size = 160
aligned_images = []
num_images_test = min(16, nrof_images)
aligned_images = np.zeros((num_images_test + 1, image_size, image_size, 3))
# print(num_images_test)
for i in range(num_images_test + 1):
if i == num_images_test:
image = imagefile
else:
# image = cv2.imread(image_paths[i])
image = misc.imread(image_paths[i])
image = misc.imresize(image, (image_size, image_size), interp='bilinear')
# scaled = cv2.resize(cropped, (input_image_size,input_image_size),interpolation=cv2.INTER_CUBIC)
scaled = prewhiten(image)
images = np.zeros((1, image_size, image_size, 3))
images[0, :, :, :] = scaled
# images = scaled.reshape(-1,image_size,image_size,3)
images = prewhiten(images)
# aligned_images.append(images)
aligned_images[i, :, :, :] = images
# aligned_images = np.stack(aligned_images)
with tf.Graph().as_default():
gpu_options = tf.GPUOptions(per_process_gpu_memory_fraction=0.5)
sess = tf.Session(config=tf.ConfigProto(gpu_options=gpu_options, log_device_placement=False))
with sess.as_default():
modeldir = "main/model/resource/face_recog/pre_model/20170511-185253.pb"
load_model(modeldir)
images_placeholder = tf.get_default_graph().get_tensor_by_name("input:0")
embeddings = tf.get_default_graph().get_tensor_by_name("embeddings:0")
phase_train_placeholder = tf.get_default_graph().get_tensor_by_name("phase_train:0")
embedding_size = embeddings.get_shape()[1]
feed_dict = {images_placeholder: aligned_images, phase_train_placeholder: False}
emb_array = sess.run(embeddings, feed_dict=feed_dict)
# print("emb_length", len(emb_array))
dist = []
for i in range(len(emb_array) - 1):
dist.append(get_embedding_distance(emb_array[num_images_test], emb_array[i]))
print(dist)
if (min(dist)) < threshold:
verification = 1
else:
verification = 0
return verification, min(dist)
def get_embedding_distance(emb1, emb2):
dist = np.sqrt(np.sum(np.square(np.subtract(emb1, emb2))))
return dist
def load_model(model):
model_exp = os.path.expanduser(model)
if (os.path.isfile(model_exp)):
print('Model filename: %s' % model_exp)
with gfile.FastGFile(model_exp, 'rb') as f:
graph_def = tf.GraphDef()
graph_def.ParseFromString(f.read())
tf.import_graph_def(graph_def, name='')
else:
print('Model directory: %s' % model_exp)
meta_file, ckpt_file = get_model_filenames(model_exp)
print('Metagraph file: %s' % meta_file)
print('Checkpoint file: %s' % ckpt_file)
saver = tf.train.import_meta_graph(os.path.join(model_exp, meta_file))
saver.restore(tf.get_default_session(), os.path.join(model_exp, ckpt_file))
def prewhiten(x):
mean = np.mean(x)
std = np.std(x)
std_adj = np.maximum(std, 1.0 / np.sqrt(x.size))
y = np.multiply(np.subtract(x, mean), 1 / std_adj)
return y
def crop(image, random_crop, image_size):
if image.shape[1] > image_size:
sz1 = int(image.shape[1] // 2)
sz2 = int(image_size // 2)
if random_crop:
diff = sz1 - sz2
(h, v) = (np.random.randint(-diff, diff + 1), np.random.randint(-diff, diff + 1))
else:
(h, v) = (0, 0)
image = image[(sz1 - sz2 + v):(sz1 + sz2 + v), (sz1 - sz2 + h):(sz1 + sz2 + h), :]
return image
def flip(image, random_flip):
if random_flip and np.random.choice([True, False]):
image = np.fliplr(image)
return image
def to_rgb(img):
w, h = img.shape
ret = np.empty((w, h, 3), dtype=np.uint8)
ret[:, :, 0] = ret[:, :, 1] = ret[:, :, 2] = img
return ret
def get_model_filenames(model_dir):
files = os.listdir(model_dir)
meta_files = [s for s in files if s.endswith('.meta')]
if len(meta_files) == 0:
raise ValueError('No meta file found in the model directory (%s)' % model_dir)
elif len(meta_files) > 1:
raise ValueError('There should not be more than one meta file in the model directory (%s)' % model_dir)
meta_file = meta_files[0]
meta_files = [s for s in files if '.ckpt' in s]
max_step = -1
for f in files:
step_str = re.match(r'(^model-[\w\- ]+.ckpt-(\d+))', f)
if step_str is not None and len(step_str.groups()) >= 2:
step = int(step_str.groups()[1])
if step > max_step:
max_step = step
ckpt_file = step_str.groups()[0]
return meta_file, ckpt_file
def print_id_dataset_table(id_dataset):
nrof_samples = len(id_dataset)
print('Images:')
for i in range(nrof_samples):
print('%1d: %s' % (i, id_dataset[i].image_path))
print('')
print('Distance matrix')
print(' ', end='')
for i in range(nrof_samples):
name = os.path.splitext(os.path.basename(id_dataset[i].name))[0]
print(' %s ' % name, end='')
print('')
for i in range(nrof_samples):
name = os.path.splitext(os.path.basename(id_dataset[i].name))[0]
print('%s ' % name, end='')
for j in range(nrof_samples):
dist = get_embedding_distance(id_dataset[i].embedding, id_dataset[j].embedding)
print(' %1.4f ' % dist, end='')
print('')
def test_run(pnet, rnet, onet, sess, images_placeholder, phase_train_placeholder, embeddings, id_dataset, test_folder):
if test_folder is None:
return
image_names = os.listdir(os.path.expanduser(test_folder))
image_paths = [os.path.join(test_folder, img) for img in image_names]
nrof_images = len(image_names)
aligned_images = []
aligned_image_paths = []
for i in range(nrof_images):
image = misc.imread(image_paths[i])
face_patches, _, _ = detect_and_align.align_image(image, pnet, rnet, onet)
aligned_images = aligned_images + face_patches
aligned_image_paths = aligned_image_paths + [image_paths[i]] * len(face_patches)
aligned_images = np.stack(aligned_images)
feed_dict = {images_placeholder: aligned_images, phase_train_placeholder: False}
embs = sess.run(embeddings, feed_dict=feed_dict)
for i in range(len(embs)):
misc.imsave('outfile' + str(i) + '.jpg', aligned_images[i])
matching_id, dist = find_matching_id(id_dataset, embs[i, :])
if matching_id:
print('Found match %s for %s! Distance: %1.4f' % (matching_id, aligned_image_paths[i], dist))
else:
print('Couldn\'t fint match for %s' % (aligned_image_paths[i]))
def load_embeddings_from_json(enrollment_json_dir):
'''
Read all json files in disk (./CDAC/userid/enrollment_data_json/)
and combine them into single dictionary
return: dictionary of json files
'''
embeddings = {} # {username:[[vector1],[vector2],..], username:[[vector1],[vector2]]....}
cls_folds = os.listdir(enrollment_json_dir)
for fold in cls_folds:
files = glob.glob(os.path.join(enrollment_json_dir, fold) + "/*.json")
embed_list = []
for f in files:
with open(f) as j_file:
embed_list.append(json.load(j_file)['data'][0]) # json_format = {data: [[embeddings]]}
embeddings[fold] = embed_list
print("SUCCESS: Embeddings loaded sunccessfully")
return embeddings
def parse_arguments(argv):
parser = argparse.ArgumentParser()
parser.add_argument('model', type=str, default="model/20170512-110547.pb",
help='Could be either a directory containing the meta_file and ckpt_file or a model protobuf (.pb) file')
parser.add_argument('id_folder', default="enrollment_data", type=str, nargs='+',
help='Folder containing ID folders')
parser.add_argument('--test_folder', type=str, help='Folder containing test images.', default=None)
return parser.parse_args(argv)
def get_aligned_face_r_detector(detector, img, thresh, do_flip, margin, input_resolution):
count = 1
im_shape = img.shape
scales = [640, 1080]
target_size = scales[0]
max_size = scales[1]
im_size_min = np.min(im_shape[0:2])
im_size_max = np.max(im_shape[0:2])
#im_scale = 1.0
#if im_size_min>target_size or im_size_max>max_size:
im_scale = float(target_size) / float(im_size_min)
# prevent bigger axis from being more than max_size:
if np.round(im_scale * im_size_max) > max_size:
im_scale = float(max_size) / float(im_size_max)
#print('im_scale', im_scale)
scales = [im_scale]
for c in range(count):
bboxes, landmarks = detector.detect(img, thresh, scales=scales, do_flip=False)
bboxes, landmarks_orig = get_largest_face_rface(bboxes, landmarks)
landmarks = prepare_landmarks_with_margin(landmarks_orig, margin, img)
aligned_face = align_face(img, landmarks[0][..., ::-1], input_resolution)
nrof_faces = 1
return nrof_faces, aligned_face, bboxes, landmarks_orig
def get_largest_face_rface(bboxes,landmarks ):
#num_faces = len(bboxes)
bounding_box_size = (bboxes[:,2]-bboxes[:,0])*(bboxes[:,3]-bboxes[:,1])
indx = np.argmax(bounding_box_size)
bboxes = bboxes[indx]
points = landmarks[indx]
landmarks = np.zeros((5,2), dtype=float)
landmarks[:,0] = points[:,1]
landmarks[:,1] = points[:,0]
landmarks = np.asarray([landmarks])
return bboxes, landmarks
def prepare_landmarks_with_margin(landmarks, margin, imagedata):
## Left Eye
landmarks[0][0][0] = np.maximum(landmarks[0][0][0] - margin/2,0) # Y Component
landmarks[0][0][1] = np.maximum(landmarks[0][0][1] - margin/2,0) # X Component
## Right Eye
landmarks[0][1][0] = np.maximum(landmarks[0][1][0] - margin/2, 0) # Y Component
landmarks[0][1][1] = np.minimum(landmarks[0][1][1] + margin/2, imagedata.shape[1]) # X Component
## Left Mouth
landmarks[0][3][0] = np.minimum(landmarks[0][3][0] + margin/2, imagedata.shape[1]) # Y Component
landmarks[0][3][1] = np.maximum(landmarks[0][3][1] - margin/2, 0) # X Component
## right Mouth
landmarks[0][4][0] = np.minimum(landmarks[0][4][0] + margin/2, imagedata.shape[1]) # Y Component
landmarks[0][4][1] = np.minimum(landmarks[0][4][1] + margin/2, imagedata.shape[1]) # X Component
return landmarks
if __name__ == '__main__':
main(parse_arguments(sys.argv[1:]))
<file_sep>/rest/api/views.py
from django.shortcuts import render
from django.http import JsonResponse, HttpResponse
from django.views.decorators.csrf import csrf_exempt
import time
from rest_framework.views import APIView
from .apps import ApiConfig
import base64
import cv2
import numpy as np
from .face.mtcnn import detect_and_align
from .face.retinaface.recog_support import get_aligned_face
from .face.retinaface.recog_support import get_embedding_rface
# Create your views here.
def get_emb_dis(emb1, emb2):
dist = np.sqrt(np.sum(np.square(np.subtract(emb1, emb2))))
return dist
@csrf_exempt
def start(request):
return JsonResponse({"Status": "Api Running"})
class model_call(APIView):
def post(self, request):
img1 = (request.POST.get('imagefile1', default=None))
input_image_size = 160
a = img1.encode("UTF-8")
b = base64.b64decode(a)
vector1 = np.array(bytearray(b), dtype='uint8')
image1 = cv2.imdecode(vector1, cv2.IMREAD_COLOR)
img2 = (request.POST.get('imagefile2', default=None))
input_image_size = 160
c = img2.encode("UTF-8")
d = base64.b64decode(c)
vector2 = np.array(bytearray(d), dtype='uint8')
image2 = cv2.imdecode(vector2, cv2.IMREAD_COLOR)
onet = ApiConfig.onet
rnet = ApiConfig.rnet
pnet = ApiConfig.pnet
#rface = ApiConfig.rface_model
detect_multiple_faces = 1
margin = 0
input_resolution = 160
num_faces1, aligned_face1 = get_aligned_face(pnet, rnet, onet, image1, detect_multiple_faces,
margin, input_resolution)
num_faces2, aligned_face2 = get_aligned_face(pnet, rnet, onet, image2, detect_multiple_faces,
margin, input_resolution)
print("num_faces1",num_faces1)
print("num_faces2", num_faces2)
#print(aligned_face2.shape)
with ApiConfig.graph_face.as_default():
with ApiConfig.sess_face.as_default():
if num_faces1 == 0:
res = {'status': False, 'message': f"Face Not Detected in 1st images", 'distance': -1}
return JsonResponse(res, safe= False)
if num_faces2 == 0:
res = {'status': False, 'message': f"Face Not Detected in 2nd images", 'distance': -1}
return JsonResponse(res, safe=False)
if num_faces1 > 1:
res = {'status': False, 'message': f"Multiple Faces Detected in 1st image", 'distance': -1}
return JsonResponse(res, safe=False)
if num_faces2 > 1:
res = {'status': False, 'message': f"Multiple Faces Detected in 2nd image", 'distance': -1}
return JsonResponse(res, safe=False)
if num_faces1 == 1 and num_faces2 == 1:
face_embedding1 = get_embedding_rface(ApiConfig.rface_model, aligned_face1)
# print(face_embedding1)
face_embedding2 = get_embedding_rface(ApiConfig.rface_model, aligned_face2)
dist = get_emb_dis(face_embedding1, face_embedding2)
dist=np.float64(dist)
if dist > 1.1:
res = {'status': False, 'message': f"Faces are of not same person", 'distance':dist}
return JsonResponse(res, safe=False)
else:
res = {'status': True, 'message': f"Faces matches", 'distance':dist}
return JsonResponse(res, safe=False)
else:
return None
<file_sep>/rest/api/apps.py
from django.apps import AppConfig
from pathlib import Path
import numpy as np
import tensorflow as tf
from keras.models import Model
from keras.layers import Lambda, Input
from .face.retinaface.rface_model.lresnet100e_ir import LResNet100E_IR
from .face.mtcnn import detect_and_align
from .face.retinaface.recog_support import resize_tensor
from .face.retinaface.recog_support import l2_norm
class ApiConfig(AppConfig):
name = 'api'
#predictor = MTCNN()
np.random.seed(1234)
# net = SupportMethods.build_rface()
## Parameters
input_resolution = 112
input_image_size = 160
margin = 0
thresh = 0.8
mask_thresh = 0.2
count = 1
gpuid = -1
# detector = RetinaFaceCoV('./models/detector/RetFaceCov/model/mnet_cov2', 0, gpuid, 'net3l')
graph_face = tf.Graph()
sess_face = tf.Session(graph=graph_face)
with graph_face.as_default():
with sess_face.as_default():
MODEL_MTCNN=Path('/home/bhatiya/web_dev/django/rest_api/api/fr/d_npy')
pnet, rnet, onet = detect_and_align.create_mtcnn(sess_face, MODEL_MTCNN)
### CReate RFACE MODEL
classes = 512
latent_dim = classes
input_resolution = 112
weights_path = "/home/bhatiya/work/dj_rest/rest/api/face_recog/rface_model/lresnet100e_ir_keras.h5"
lresnet100e_ir = LResNet100E_IR(weights_path=weights_path)
input_tensor = Input((None, None, 3))
resize_layer = resize_tensor(size=input_resolution)
l2_normalize = l2_norm(latent_dim)
output_tensor = l2_normalize(lresnet100e_ir(resize_layer(input_tensor)))
rface_model = Model(input_tensor, output_tensor)
rface_model.trainable = False
<file_sep>/rest/api/face/retinaface/face_detection/face_detection_in_background.py
""" Imports the controller """
from __future__ import division
from __future__ import absolute_import
from __future__ import print_function
import os
import sys
from main.model.resource.Resources import SupportMethods
import numpy as np
from scipy import misc
import shutil
import tensorflow as tf
from main.model.resource.face_detection import detect_and_align
from main.model.resource.face_recog import recog_support
import csv
import json
srcpath = 'main/data/temp/'
json_path = 'main/data/temp2/'
detect_multiple_faces = 0
with tf.Graph().as_default():
with tf.Session() as sess:
margin = 44
image_size = 160
pnet, rnet, onet = detect_and_align.create_mtcnn(sess, None)
n = 1
while n==1:
imagelist = os.listdir(srcpath)
for imagefile in imagelist:
try:
image = misc.imread(os.path.join(srcpath, imagefile))
if image.ndim == 2:
image = SupportMethods().to_rgb(image)
image = image[:, :, 0:3]
bounding_boxes, landmarks, scores = detect_and_align.detect_face(image, pnet, rnet, onet)
#print(landmarks)
nrof_bb = bounding_boxes.shape[0]
if nrof_bb > 0:
landmark = []
det = bounding_boxes[:, 0:4]
det_arr = []
img_size = np.asarray(image.shape)[0:2]
if nrof_bb > 1:
if detect_multiple_faces:
for i in range(nrof_bb):
det_arr.append(np.squeeze(det[i]))
for i in range(10):
landmark.append(np.squeeze(landmarks[i]))
if len(landmark) > 0:
for i in range(len(landmark[0])):
for j in range(10):
landmark1.append(int(landmark[j][i]))
else:
bounding_box_size = (det[:, 2] - det[:, 0]) * (det[:, 3] - det[:, 1])
img_center = img_size / 2
offsets = np.vstack([(det[:, 0] + det[:, 2]) / 2 - img_center[1],
(det[:, 1] + det[:, 3]) / 2 - img_center[0]])
offset_dist_squared = np.sum(np.power(offsets, 2.0), 0)
index = np.argmax(
bounding_box_size - offset_dist_squared * 2.0) # some extra weight on the centering
det_arr.append(det[index, :])
landmark.append(np.squeeze(landmarks[index, :]))
landmark1 = [int(land) for land in landmark[0]]
else:
det_arr.append(np.squeeze(det))
landmark.append(np.squeeze(landmarks))
landmark1 = [int(land) for land in landmark[0]]
#print(landmark)
output = []
for i in range(len(det_arr)):
for j in range(4):
output.append(det_arr[i][j])
record = {'Bounding_box': output,
'Landmarks': landmark1
}
else:
record = {'Bounding_box': 'No',
'Landmarks': 'No'
}
print(record)
filename = os.path.join(json_path, os.path.splitext(imagefile)[0] + '.json')
with open(filename, 'w') as f1:
json.dump(record, f1)
os.remove(os.path.join(srcpath, imagefile))
#shutil.move(os.path.join(srcpath, imagefile), 'main/data/temp2/'+imagefile)
except:
pass
<file_sep>/rest/api/face/retinaface/recog_support_bkp.py
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
from keras.layers import Lambda, Input
from keras.models import Model
from keras import backend as K
from main.model.resource.face_recog.rface_model.lresnet100e_ir import LResNet100E_IR
import tensorflow as tf
from tensorflow.python.platform import gfile
import numpy as np
import sys
import os
from main.model.resource.face_detection import detect_and_align
from main.model.resource.face_recog import id_data
from scipy import misc
import re
import cv2
import time
import pickle
from scipy import misc
import json
import glob
def load_data(image_paths, do_random_crop, do_random_flip, image_size, do_prewhiten=True):
nrof_samples = len(image_paths)
images = np.zeros((nrof_samples, image_size, image_size, 3))
for i in range(nrof_samples):
img = misc.imread(image_paths[i])
if img.ndim == 2:
img = to_rgb(img)
if do_prewhiten:
img = prewhiten(img)
img = crop(img, do_random_crop, image_size)
img = flip(img, do_random_flip)
images[i,:,:,:] = img
return images
def find_matching_id(id_dataset, embedding):
threshold = 0.65
min_dist = 10.0
matching_id = None
for id_data in id_dataset:
dist = get_embedding_distance(id_data.embedding, embedding)
if dist < threshold and dist < min_dist:
min_dist = dist
matching_id = id_data.name
return matching_id, min_dist
def get_embedding(sess, imgfile):
images_placeholder = tf.get_default_graph().get_tensor_by_name("input:0")
embeddings = tf.get_default_graph().get_tensor_by_name("embeddings:0")
phase_train_placeholder = tf.get_default_graph().get_tensor_by_name("phase_train:0")
embedding_size = 128
image_size = 160
emb_array = np.zeros((1, embedding_size))
#cropped = flip(imgfile, False)
#scaled= misc.imresize(cropped, (image_size, image_size), interp='bilinear')
#scaled = cv2.resize(cropped, (input_image_size,input_image_size),interpolation=cv2.INTER_CUBIC)
images = np.zeros((1, image_size, image_size, 3))
img = prewhiten(imgfile)
images[0,:,:,:] = img
images = prewhiten(images)
feed_dict = {images_placeholder: images, phase_train_placeholder: False}
emb_array = sess.run(embeddings, feed_dict=feed_dict)
return emb_array
def get_embedding_rface(net, imgfile):
'''
imgfile: image numpy narray
'''
embedding = net.predict([imgfile]) #embedding = [[feature_vectors]]
return embedding
def resize_tensor(size):
'''
load_rface_model() supporting function
size: input image size of model
'''
input_tensor = Input((None, None, 3))
output_tensor = Lambda(lambda x: tf.image.resize_bilinear(x, [size, size]))(input_tensor)
return Model(input_tensor, output_tensor)
def l2_norm(latent_dim):
'''
load_rface_model() supporting function
'''
input_tensor = Input((latent_dim,))
output_tensor = Lambda(lambda x: K.l2_normalize(x))(input_tensor)
return Model(input_tensor, output_tensor)
def load_rface_model():
classes = 512
latent_dim = classes
input_resolution = 112
weights_path = "main/model/resource/face_recog/rface_model/lresnet100e_ir_keras.h5"
lresnet100e_ir = LResNet100E_IR(weights_path=weights_path)
input_tensor = Input((None, None, 3))
resize_layer = resize_tensor(size=input_resolution)
l2_normalize = l2_norm(latent_dim)
output_tensor = l2_normalize(lresnet100e_ir(resize_layer(input_tensor)))
net = Model(input_tensor, output_tensor)
net.trainable = False
print("Network Built...")
return net
def get_face_id_new(emb_array, model, class_names):
predictions = model.predict_proba(emb_array)
#print(predictions)
best_class_indices = np.argmax(predictions, axis=1)
#print(best_class_indices)
best_class_probabilities = predictions[np.arange(len(best_class_indices)), best_class_indices]
best_class_probability = best_class_probabilities[0]
best_class_indice = best_class_indices[0]
#print(best_class_probabilities)
print("Best class Detected, Probobility", class_names[best_class_indice], best_class_probability)
matching_id = class_names[best_class_indice]
'''
if best_class_probability > 0.35:
matching_id = class_names[best_class_indice]
else:
matching_id = 'Unknown'
'''
return matching_id
def verify_same_class_photos(tempenrollmentfolder_json, threshold=0.80):
#threshold = 1.0
testfolder = tempenrollmentfolder_json
file_names = os.listdir(testfolder)
num_images_test = len(file_names)
print(tempenrollmentfolder_json, num_images_test)
dist = []
for i in range(num_images_test-1):
filepath1 = os.path.join(testfolder, file_names[i])
with open(filepath1, 'r') as f1:
result1 = json.load(f1)
edata1 = result1['data']
for j in range(i+1, num_images_test, 1):
filepath2 = os.path.join(testfolder, file_names[j])
with open(filepath2, 'r') as f2:
result2 = json.load(f2)
edata2 = result2['data']
dist.append(get_embedding_distance(edata1, edata2))
max_dist = max(np.array(dist))
if max_dist < threshold:
verification = 1
else:
verification = 0
return verification, dist
def verification_user(emb_array, enrollfolder_JSON_path, threshold ):
'''
loads emebeddings from ./enrollment_data_json/class_id/ directory and
then calculates distance.
enrollfolder_JSON_path: full path to class id whose embeddings are to be matched with emb_array.
'''
#0.6
count = 0
dist_all = []
for filename in os.listdir(enrollfolder_JSON_path):
filepath = os.path.join(enrollfolder_JSON_path, filename)
print(filepath)
with open(filepath, 'r') as f1:
result = json.load(f1)
edata = result['data']
dist= get_embedding_distance(edata, emb_array)
dist_all.append(dist)
if dist < threshold:
count += 1
if count > 0: #1 # check if >0 images match then verification status will be 1
verification = 1
else:
verification = 0
return verification, dist_all
def verification_user_from_memoryEmbeddings(emb_array, cls_embeddings):
'''
find distance of passed emb_array with memory loaded embeddings (dictionary of embeddings)
emb_array: image features
cls_embeddings: list of embeddings for user to be verified
return: verification status and dist of emb_array with matching embedding in list.
'''
threshold = 0.75 #0.6
count = 0
dist_all = []
for embeds in cls_embeddings:
edata = embeds
dist= get_embedding_distance(edata, emb_array)
dist_all.append(dist)
if dist < threshold:
count += 1
if count > 0: #1 # check if >0 images match then verification status will be 1
verification = 1
else:
verification = 0
return verification, dist_all
def verify_id(emb_array, enrollfolder_JSON_path,threshold=0.66):
# threshold = 0.66 #0.73
#testfolder = "main/data/enrollment_data_json/"
testfolder = enrollfolder_JSON_path
folders = os.listdir(testfolder)
dist = []
classes = []
for fold in folders:
file_names = os.listdir(os.path.join(testfolder,fold))
num_images_test = min(8, len(file_names))
for i in range(num_images_test):
filepath = os.path.join(testfolder, fold, file_names[i])
with open(filepath, 'r') as f1:
try:
result = json.load(f1)
except Exception as e:
print(e)
print(filepath)
edata = result['data']
dist.append(get_embedding_distance(edata, emb_array))
classes.append(fold)
#dist = np.array(dist)
min_dist = min(np.array(dist))
min_index = dist.index(min_dist)
class_id = classes[min_index]
verification = 0
if min_dist <= threshold:
verification = 1
else:
verification = 0
return verification, min(dist), class_id
def verify_id_json(emb_array, embeddings):
'''
author - Ajay
compares given embeddings with embeddings dictionay
emb_array: Embedding vector of an image
embeddings: pre-loaded embeddings dictionary of all users
return : verification status, min distance, class id matched with
'''
threshold = 0.75 #0.55
dist = []
classes = []
for fold in embeddings.keys():
embeds_list = embeddings[fold]
nrof_images = len(embeds_list)
for i in range(nrof_images):
edata = embeds_list[i]
dist.append(get_embedding_distance(edata, emb_array))
classes.append(fold)
#print(dist)
#dist = np.array(dist)
min_dist = min(np.array(dist))
min_index = dist.index(min_dist)
class_id = classes[min_index]
print("Minimum dist with class: ",class_id)
if min_dist < threshold:
verification = 1
else:
verification = 0
return verification, min(dist), class_id
def identify_verify_id_new(emb_array, userid):
'''
used for BSID
'''
threshold = 0.73
testfolder = "main/data/enrollment_data_json/" + str(userid)
file_names = os.listdir(testfolder)
#file_paths = [os.path.join(testfolder, img) for img in image_names]
num_images_test = min(16, len(file_names))
dist = []
for i in range(num_images_test):
filepath = os.path.join(testfolder, file_names[i])
with open(filepath, 'r') as f1:
result = json.load(f1)
edata = result['data']
dist.append(get_embedding_distance(edata, emb_array))
#print(dist)
if (min(dist)) < threshold:
verification = 1
else:
verification = 0
return verification, min(dist)
def get_face_id(imgfile):
classifier_filename = "main/model/resource/classifier/SVMclassifier.pkl"
with open(classifier_filename, 'rb') as infile:
(model, class_names) = pickle.load(infile)
with tf.Graph().as_default():
gpu_options = tf.GPUOptions(per_process_gpu_memory_fraction=0.5)
sess = tf.Session(config=tf.ConfigProto(gpu_options=gpu_options, log_device_placement=False))
with sess.as_default():
modeldir = "main/model/resource/face_recog/pre_model/20170511-185253.pb"
load_model(modeldir)
images_placeholder = tf.get_default_graph().get_tensor_by_name("input:0")
embeddings = tf.get_default_graph().get_tensor_by_name("embeddings:0")
phase_train_placeholder = tf.get_default_graph().get_tensor_by_name("phase_train:0")
embedding_size = embeddings.get_shape()[1]
image_size = 160
emb_array = np.zeros((1, embedding_size))
#cropped = flip(imgfile, False)
#scaled= misc.imresize(cropped, (image_size, image_size), interp='bilinear')
#scaled = cv2.resize(cropped, (input_image_size,input_image_size),interpolation=cv2.INTER_CUBIC)
images = np.zeros((1, image_size, image_size, 3))
img = prewhiten(imgfile)
images[0,:,:,:] = img
images = prewhiten(images)
feed_dict = {images_placeholder: images, phase_train_placeholder: False}
emb_array = sess.run(embeddings, feed_dict=feed_dict)
predictions = model.predict_proba(emb_array)
#print(predictions)
best_class_indices = np.argmax(predictions, axis=1)
#print(best_class_indices)
best_class_probabilities = predictions[np.arange(len(best_class_indices)), best_class_indices]
best_class_probability = best_class_probabilities[0]
best_class_indice = best_class_indices[0]
#print(best_class_probabilities)
print(class_names[best_class_indice], best_class_probability)
matching_id = class_names[best_class_indice]
'''
if best_class_probability > 0.35:
matching_id = class_names[best_class_indice]
else:
matching_id = 'Unknown'
'''
return matching_id
def identify_verify_id(imagefile, userid):
'''
for BSID
'''
threshold = 0.80
print(userid)
testfolder = "main/data/enrollment_data/" + str(userid)
image_names = os.listdir(testfolder)
image_paths = [os.path.join(testfolder, img) for img in image_names]
nrof_images = len(image_names)
image_size = 160
aligned_images = []
num_images_test = min(16, nrof_images)
aligned_images = np.zeros((num_images_test+1, image_size, image_size, 3))
#print(num_images_test)
for i in range(num_images_test+1):
if i == num_images_test:
image = imagefile
else:
#image = cv2.imread(image_paths[i])
image = misc.imread(image_paths[i])
image= misc.imresize(image, (image_size, image_size), interp='bilinear')
#scaled = cv2.resize(cropped, (input_image_size,input_image_size),interpolation=cv2.INTER_CUBIC)
scaled = prewhiten(image)
images = np.zeros((1, image_size, image_size, 3))
images[0,:,:,:] = scaled
#images = scaled.reshape(-1,image_size,image_size,3)
images = prewhiten(images)
#aligned_images.append(images)
aligned_images[i,:,:,:] = images
#aligned_images = np.stack(aligned_images)
with tf.Graph().as_default():
gpu_options = tf.GPUOptions(per_process_gpu_memory_fraction=0.5)
sess = tf.Session(config=tf.ConfigProto(gpu_options=gpu_options, log_device_placement=False))
with sess.as_default():
modeldir = "main/model/resource/face_recog/pre_model/20170511-185253.pb"
load_model(modeldir)
images_placeholder = tf.get_default_graph().get_tensor_by_name("input:0")
embeddings = tf.get_default_graph().get_tensor_by_name("embeddings:0")
phase_train_placeholder = tf.get_default_graph().get_tensor_by_name("phase_train:0")
embedding_size = embeddings.get_shape()[1]
feed_dict = {images_placeholder: aligned_images, phase_train_placeholder: False}
emb_array = sess.run(embeddings, feed_dict=feed_dict)
#print("emb_length", len(emb_array))
dist = []
for i in range(len(emb_array)-1):
dist.append(get_embedding_distance(emb_array[num_images_test], emb_array[i]))
print(dist)
if (min(dist)) < threshold:
verification = 1
else:
verification = 0
return verification, min(dist)
def get_embedding_distance(emb1, emb2):
dist = np.sqrt(np.sum(np.square(np.subtract(emb1, emb2))))
return dist
def load_model(model):
model_exp = os.path.expanduser(model)
if (os.path.isfile(model_exp)):
print('Model filename: %s' % model_exp)
with gfile.FastGFile(model_exp, 'rb') as f:
graph_def = tf.GraphDef()
graph_def.ParseFromString(f.read())
tf.import_graph_def(graph_def, name='')
else:
print('Model directory: %s' % model_exp)
meta_file, ckpt_file = get_model_filenames(model_exp)
print('Metagraph file: %s' % meta_file)
print('Checkpoint file: %s' % ckpt_file)
saver = tf.train.import_meta_graph(os.path.join(model_exp, meta_file))
saver.restore(tf.get_default_session(), os.path.join(model_exp, ckpt_file))
def prewhiten(x):
mean = np.mean(x)
std = np.std(x)
std_adj = np.maximum(std, 1.0/np.sqrt(x.size))
y = np.multiply(np.subtract(x, mean), 1/std_adj)
return y
def crop(image, random_crop, image_size):
if image.shape[1]>image_size:
sz1 = int(image.shape[1]//2)
sz2 = int(image_size//2)
if random_crop:
diff = sz1-sz2
(h, v) = (np.random.randint(-diff, diff+1), np.random.randint(-diff, diff+1))
else:
(h, v) = (0,0)
image = image[(sz1-sz2+v):(sz1+sz2+v),(sz1-sz2+h):(sz1+sz2+h),:]
return image
def flip(image, random_flip):
if random_flip and np.random.choice([True, False]):
image = np.fliplr(image)
return image
def to_rgb(img):
w, h = img.shape
ret = np.empty((w, h, 3), dtype=np.uint8)
ret[:, :, 0] = ret[:, :, 1] = ret[:, :, 2] = img
return ret
def get_model_filenames(model_dir):
files = os.listdir(model_dir)
meta_files = [s for s in files if s.endswith('.meta')]
if len(meta_files) == 0:
raise ValueError('No meta file found in the model directory (%s)' % model_dir)
elif len(meta_files) > 1:
raise ValueError('There should not be more than one meta file in the model directory (%s)' % model_dir)
meta_file = meta_files[0]
meta_files = [s for s in files if '.ckpt' in s]
max_step = -1
for f in files:
step_str = re.match(r'(^model-[\w\- ]+.ckpt-(\d+))', f)
if step_str is not None and len(step_str.groups()) >= 2:
step = int(step_str.groups()[1])
if step > max_step:
max_step = step
ckpt_file = step_str.groups()[0]
return meta_file, ckpt_file
def print_id_dataset_table(id_dataset):
nrof_samples = len(id_dataset)
print('Images:')
for i in range(nrof_samples):
print('%1d: %s' % (i, id_dataset[i].image_path))
print('')
print('Distance matrix')
print(' ', end='')
for i in range(nrof_samples):
name = os.path.splitext(os.path.basename(id_dataset[i].name))[0]
print(' %s ' % name, end='')
print('')
for i in range(nrof_samples):
name = os.path.splitext(os.path.basename(id_dataset[i].name))[0]
print('%s ' % name, end='')
for j in range(nrof_samples):
dist = get_embedding_distance(id_dataset[i].embedding, id_dataset[j].embedding)
print(' %1.4f ' % dist, end='')
print('')
def test_run(pnet, rnet, onet, sess, images_placeholder, phase_train_placeholder, embeddings, id_dataset, test_folder):
if test_folder is None:
return
image_names = os.listdir(os.path.expanduser(test_folder))
image_paths = [os.path.join(test_folder, img) for img in image_names]
nrof_images = len(image_names)
aligned_images = []
aligned_image_paths = []
for i in range(nrof_images):
image = misc.imread(image_paths[i])
face_patches, _, _ = detect_and_align.align_image(image, pnet, rnet, onet)
aligned_images = aligned_images + face_patches
aligned_image_paths = aligned_image_paths + [image_paths[i]] * len(face_patches)
aligned_images = np.stack(aligned_images)
feed_dict = {images_placeholder: aligned_images, phase_train_placeholder: False}
embs = sess.run(embeddings, feed_dict=feed_dict)
for i in range(len(embs)):
misc.imsave('outfile' + str(i) + '.jpg', aligned_images[i])
matching_id, dist = find_matching_id(id_dataset, embs[i, :])
if matching_id:
print('Found match %s for %s! Distance: %1.4f' % (matching_id, aligned_image_paths[i], dist))
else:
print('Couldn\'t fint match for %s' % (aligned_image_paths[i]))
def load_embeddings_from_json(enrollment_json_dir):
'''
Read all json files in disk (./CDAC/userid/enrollment_data_json/)
and combine them into single dictionary
return: dictionary of json files
'''
embeddings = {} # {username:[[vector1],[vector2],..], username:[[vector1],[vector2]]....}
cls_folds = os.listdir(enrollment_json_dir)
for fold in cls_folds:
files = glob.glob(os.path.join(enrollment_json_dir,fold)+"/*.json")
embed_list = []
for f in files:
with open(f) as j_file:
embed_list.append(json.load(j_file)['data'][0]) #json_format = {data: [[embeddings]]}
embeddings[fold] = embed_list
print("SUCCESS: Embeddings loaded sunccessfully")
return embeddings
def parse_arguments(argv):
parser = argparse.ArgumentParser()
parser.add_argument('model', type=str, default="model/20170512-110547.pb", help='Could be either a directory containing the meta_file and ckpt_file or a model protobuf (.pb) file')
parser.add_argument('id_folder', default="enrollment_data", type=str, nargs='+', help='Folder containing ID folders')
parser.add_argument('--test_folder', type=str, help='Folder containing test images.', default=None)
return parser.parse_args(argv)
if __name__ == '__main__':
main(parse_arguments(sys.argv[1:]))
<file_sep>/rest/api/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.start, name='home'),
path('model/', views.model_call.as_view() ),
]
<file_sep>/requirements.txt
absl-py==0.11.0
apturl==0.5.2
asgiref==3.3.1
asn1crypto==0.24.0
astor==0.8.1
astroid==2.4.2
astunparse==1.6.3
Brlapi==0.6.6
cachetools==4.2.1
certifi==2018.1.18
chardet==3.0.4
command-not-found==0.3
cryptography==2.1.4
cupshelpers==1.0
cx-Oracle==8.1.0
cycler==0.10.0
dataclasses==0.8
defer==1.0.6
distro==1.5.0
distro-info===0.18ubuntu0.18.04.1
Django==3.1.5
djangorestframework==3.12.2
facenet==1.0.5
flatbuffers==1.12
gast==0.3.3
google-auth==1.24.0
google-auth-oauthlib==0.4.2
google-pasta==0.2.0
grpcio==1.32.0
h5py==2.10.0
httplib2==0.9.2
idna==2.6
importlib-metadata==3.4.0
imutils==0.5.3
isort==5.7.0
joblib==1.0.0
Keras==2.2.3
Keras-Applications==1.0.8
Keras-Preprocessing==1.1.2
keyring==10.6.0
keyrings.alt==3.0
kiwisolver==1.3.1
language-selector==0.1
launchpadlib==1.10.6
lazr.restfulclient==0.13.5
lazr.uri==1.0.3
lazy-object-proxy==1.4.3
louis==3.5.0
macaroonbakery==1.1.3
Mako==1.0.7
Markdown==3.3.3
MarkupSafe==1.0
matplotlib==3.3.4
mccabe==0.6.1
mediapipe==0.8.2
netifaces==0.10.4
numpy==1.19.3
oauth==1.0.1
oauthlib==3.1.0
olefile==0.45.1
opencv-python==4.0.1.23
opt-einsum==3.3.0
packaging==20.4
pexpect==4.2.1
Pillow==8.1.0
protobuf==3.14.0
psutil==5.8.0
psycopg2-binary==2.8.6
pyasn1==0.4.8
pyasn1-modules==0.2.8
pycairo==1.16.2
pycrypto==2.6.1
pycups==1.9.73
pygobject==3.26.1
pylint==2.6.0
pymacaroons==0.13.0
PyNaCl==1.1.2
pyparsing==2.4.7
pyRFC3339==1.0
python-apt==1.6.5+ubuntu0.5
python-dateutil==2.6.1
python-debian==0.1.32
pytz==2018.3
pyxdg==0.25
PyYAML==3.12
reportlab==3.4.0
requests==2.25.1
requests-oauthlib==1.3.0
requests-unixsocket==0.1.5
rsa==4.7
scikit-build==0.11.1
scikit-learn==0.24.1
scipy==1.5.4
SecretStorage==2.3.1
simplejson==3.13.2
six==1.15.0
sqlparse==0.4.1
system-service==0.3
systemd-python==234
tensorboard==1.14.0
tensorboard-plugin-wit==1.8.0
tensorflow==1.14.0
tensorflow-estimator==1.14.0
termcolor==1.1.0
threadpoolctl==2.1.0
toml==0.10.2
typed-ast==1.4.2
typing-extensions==3.7.4.3
ubuntu-drivers-common==0.0.0
ufw==0.36
unattended-upgrades==0.1
urllib3==1.22
usb-creator==0.3.3
virtualenv==15.1.0
wadllib==1.3.2
Werkzeug==1.0.1
wrapt==1.12.1
xkit==0.0.0
zipp==3.4.0
zope.interface==4.3.2
<file_sep>/rest/api/face/mtcnn/enroll_user.py
import cv2
import os
import time
no_enroll_images = 15
enroll_dir = "enrollment_data"
enroll_user = "Akbarq"
enroll_path = enroll_dir + "/" + enroll_user + "/"
if not os.path.isdir(enroll_path):
os.mkdir(enroll_path)
cap = cv2.VideoCapture(0)
num = 1
while(True):
ret, frame = cap.read()
rgb = cv2.cvtColor(frame, cv2.COLOR_BGR2BGRA)
cv2.imshow('frame', rgb)
time.sleep(0.1)
if cv2.waitKey(1) & 0xFF == ord('q'):
if num <= no_enroll_images:
out = cv2.imwrite(enroll_path + str(num) + '_capture.jpg', frame)
num += 1
else:
break
cap.release()
cv2.destroyAllWindows() | 01697000908a409a0d578f2c32bf391ca67a97c6 | [
"Python",
"Text"
] | 8 | Python | bhatiyaarp/restapi_django | 34b2209996e5140ce1abac2d56e531e9ecebad5f | 863ca03b05609ea36a47bda675a98d1f08303708 |
refs/heads/master | <file_sep>
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class login
*/
@WebServlet("/login")
public class Login extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Login() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
response.getWriter().append("Served at: ").append(request.getContextPath());
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String userName = request.getParameter("userName");
String userPwd = request.getParameter("userPwd");
List<User> usuarios = new ArrayList();
boolean encontrado = false;
for(int i=1; i<=9;i++) {
User usuario = new User();
usuario.setUserName(Integer.toString(i));
usuario.setUserPwd(Integer.toString(i));
usuarios.add(usuario);
}
for(int i=0; i<usuarios.size(); i++) {
User usuario1 = usuarios.get(i);
if(userName.equals(usuario1.getUserName()) && userPwd.equals(usuario1.getUserPwd())) {
encontrado = true;
response.sendRedirect("home.jsp");
}
}
if(!encontrado) {
response.sendRedirect("loginError.jsp");
}
}
}
| 20b524aac5b8632153637fade9fc09f9e57bdeec | [
"Java"
] | 1 | Java | jasminjaramillo/Trabajos-Calidad | 6f942f99ed13ecaaf33b63475a2da6aed4f8b996 | 34b3ecb074605a518dc9d2305df6496853d6b829 |
refs/heads/master | <repo_name>Chenoachem/Updated_Pawsey_Scripts<file_sep>/Slurm.template
#!/bin/bash -l
# Calibrate the data
#SBATCH -M 'magnus'
#SBATCH --account=pawsey0280
#SBATCH -p workq
#SBATCH --time=24:00:00
#SBATCH --output=/astro/mwasci/ctremblay/Out_Files/OUTPUT.o%j
#SBATCH --error=/astro/mwasci/ctremblay/Out_Files/OUTPUT.e%j
#SBATCH --export=NONE
# Queues up some data grabbing via ASVO
#SBATCH -p copyq
#SBATCH --account=mwasci
#SBATCH --time=12:00:00
#SBATCH --output=DATADIR/Out_Files/OUTPUT.o%j
#SBATCH --error=DATADIR/Out_Files/OUTPUT.e%j
#SBATCH --export=NONE
#SBATCH -M zeus
<file_sep>/SelfCal_HydA.tmp
#!/bin/bash -l
# Calibrate the data
#SBATCH --account=pawsey0280
#SBATCH -M magnus
#SBATCH -p workq
#SBATCH --time=24:00:00
#SBATCH --output=/astro/mwasci/ctremblay/Out_Files/self_1198861104.o%j
#SBATCH --error=/astro/mwasci/ctremblay/Out_Files/self_1198861104.e%j
#SBATCH --export=NONE
#aprun="aprun -n 1 -d 20 -q "
datadir=/astro/mwasci/ctremblay
proj=G0018_VELA_Test
obsnum=1198861104
ncpus=20
# you could modify the submission script and template to change these more dynamically:
imsize=4000
scale=0.0032
# setup a clean environment
source /group/mwa/software/module-reset.sh
module use /group/mwa/software/modulefiles
module load mwapy
module load manta-ray-client
module load MWA_Tools
module list
#cd /${datadir}/${proj}/${obsnum}/Calibrators
cd /astro/mwasci/ctremblay/G0018_VELA_Test/1198861104
# Initial clean to first negative
wsclean -name ${obsnum}_initial -size ${imsize} ${imsize} -scale ${scale} -abs-mem 32 -niter 400000 -threshold 0.01 -pol I -weight briggs -1.0 ${obsnum}.ms
# FT the Stokes I model into the visibilities
wsclean -predict -name ${obsnum}_initial -abs-mem 32 -size ${imsize} ${imsize} -pol I -weight briggs -1.0 -scale ${scale} ${obsnum}.ms
# Calibrate: by default, it will use the MODEL column, which now has the FT'd self-cal model
calibrate -absmem 32 -minuv 20 ${obsnum}.ms ${obsnum}_self_solutions.bin
# Apply the calibration solutions
applysolutions ${obsnum}.ms ${obsnum}_self_solutions.bin
#Make Test Images
time wsclean -name ${obsnum}_afterself -size ${imsize} ${imsize} -abs-mem 32 -niter 400000 -auto-threshold 5 -mgain 0.85 -weight briggs -1.0 -scale ${scale} -cleanborder 1 ${obsnum}.ms
| f1f307d6ac20a73a492fd9a1c795e9fe94a99e37 | [
"Shell"
] | 2 | Shell | Chenoachem/Updated_Pawsey_Scripts | 17eda7c72bb60510b4e1c0d71ff187db64381f49 | e4c6c132e11c061005bff3501e45847dc66372e4 |
refs/heads/master | <repo_name>rrrafa/Pupilometria<file_sep>/src/pupila/Grava.java
package pupila;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import javax.swing.JOptionPane;
public class Grava {
private String vetorTab="",arquivo;
public void Gravar(String nome,String arq, double[] suave) {
for (int i=0; i<suave.length; i++) {
if (i<(suave.length-1)) {
vetorTab += suave[i]+"\t";
}else {
vetorTab += suave[i];
}
}
arquivo = arq+"\\"+nome+".txt";
try {
File f = new File(arquivo);
FileWriter fw = new FileWriter(f,false);
BufferedWriter bw = new BufferedWriter(fw);
bw.write(vetorTab);
bw.flush();
bw.close();
JOptionPane.showMessageDialog(null, "Vetor tratado salvo como:\n"+arquivo,"Processo finalizado",JOptionPane.INFORMATION_MESSAGE);
}catch (Exception e) {
JOptionPane.showMessageDialog(null, e, "ERRO", JOptionPane.ERROR_MESSAGE);
}
}
public String getVetorTab() {
return vetorTab;
}
}
<file_sep>/README.md
# Pupilometria
Medição de variação da dilatação de pupilas
<file_sep>/src/pupila/Janela.java
package pupila;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.ButtonGroup;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
import javax.swing.JTextField;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
import javax.swing.border.TitledBorder;
import javax.swing.JSeparator;
import javax.swing.JEditorPane;
import java.awt.Font;
public class Janela {
private JFrame frmD;
public JTextField txtKjh;
private JTextField textField_1;
private ButtonGroup group;
/**
* Launch the application.
*/
public static void main(String[] args) {
try {
// Set cross-platform Java L&F (also called "Metal")
UIManager.setLookAndFeel(
UIManager.getSystemLookAndFeelClassName());
}
catch (UnsupportedLookAndFeelException e) {
// handle exception
}
catch (ClassNotFoundException e) {
// handle exception
}
catch (InstantiationException e) {
// handle exception
}
catch (IllegalAccessException e) {
// handle exception
}
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Janela window = new Janela();
window.frmD.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the application.
*/
public Janela() throws Exception{
initialize();
}
/**
* Initialize the contents of the frame.
*/
private void initialize() throws Exception {
frmD = new JFrame();
frmD.setResizable(false);
frmD.setTitle("Pupilometria - Tratamento de resultados");
frmD.setBounds(100, 100, 537, 457);
frmD.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frmD.getContentPane().setLayout(null);
JButton btnNewButton = new JButton("Buscar...");
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
LeitorTXT leitura = new LeitorTXT();
try {leitura.LerOlho(0);}catch(Exception e) {}
txtKjh.setText(leitura.getArq());
}
});
btnNewButton.setBounds(304, 34, 77, 23);
frmD.getContentPane().add(btnNewButton);
txtKjh = new JTextField();
txtKjh.setText("");
txtKjh.setEditable(false);
txtKjh.setBounds(10, 35, 282, 20);
frmD.getContentPane().add(txtKjh);
txtKjh.setColumns(10);
JLabel lblLocalDoArquivo = new JLabel("Arquivo de dados original:");
lblLocalDoArquivo.setBounds(10, 11, 245, 23);
frmD.getContentPane().add(lblLocalDoArquivo);
JPanel panel = new JPanel();
panel.setBorder(new TitledBorder(null, "Olho examinado", TitledBorder.LEADING, TitledBorder.TOP, null, null));
panel.setBounds(402, 29, 116, 84);
frmD.getContentPane().add(panel);
panel.setLayout(null);
JRadioButton rdbtnDireito = new JRadioButton("Direito");
rdbtnDireito.setSelected(true);
rdbtnDireito.setBounds(6, 23, 57, 23);
panel.add(rdbtnDireito);
JRadioButton rdbtnEsquerdo = new JRadioButton("Esquerdo");
rdbtnEsquerdo.setSelected(false);
rdbtnEsquerdo.setBounds(6, 54, 71, 23);
panel.add(rdbtnEsquerdo);
group = new ButtonGroup();
group.add(rdbtnDireito);
group.add(rdbtnEsquerdo);
textField_1 = new JTextField();
textField_1.setBounds(10, 388, 508, 23);
frmD.getContentPane().add(textField_1);
textField_1.setColumns(10);
JLabel lblVetorCorrigidoseparado = new JLabel("Vetor corrigido (separado por tabula\u00E7\u00F5es):");
lblVetorCorrigidoseparado.setBounds(10, 363, 211, 14);
frmD.getContentPane().add(lblVetorCorrigidoseparado);
JButton btnExecutar = new JButton("EXECUTAR LIMPEZA");
btnExecutar.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
LeitorTXT le = new LeitorTXT();
Limpeza lim = new Limpeza();
Grava grv = new Grava();
le.mediaLargAlt();
lim.Limpa();
lim.Suaviza();
grv.Gravar("vetor",le.getPasta(),lim.getSuave());
textField_1.setText(grv.getVetorTab());
textField_1.selectAll();
textField_1.copy();
}
});
btnExecutar.setBounds(10, 80, 231, 33);
frmD.getContentPane().add(btnExecutar);
JButton btnSalvar = new JButton("SALVAR");
btnSalvar.setBounds(251, 80, 130, 33);
frmD.getContentPane().add(btnSalvar);
JSeparator separator = new JSeparator();
separator.setBounds(10, 135, 511, 20);
frmD.getContentPane().add(separator);
JEditorPane editorPane = new JEditorPane();
editorPane.setBounds(10, 191, 238, 146);
frmD.getContentPane().add(editorPane);
JEditorPane editorPane_1 = new JEditorPane();
editorPane_1.setBounds(275, 191, 231, 146);
frmD.getContentPane().add(editorPane_1);
JLabel lblOriginal = new JLabel("ORIGINAL");
lblOriginal.setFont(new Font("Tahoma", Font.BOLD, 11));
lblOriginal.setBounds(10, 166, 77, 14);
frmD.getContentPane().add(lblOriginal);
JLabel lblCorrigido = new JLabel("CORRIGIDO");
lblCorrigido.setFont(new Font("Tahoma", Font.BOLD, 11));
lblCorrigido.setBounds(275, 166, 84, 14);
frmD.getContentPane().add(lblCorrigido);
}
public void setTxt(String txt) {
txtKjh.setText(txt);
JOptionPane.showMessageDialog(null, txt);
}
}
| a90e7cd1ecaf4b25466317b36417429a0226830f | [
"Markdown",
"Java"
] | 3 | Java | rrrafa/Pupilometria | 51a60047281c394c0850b44441f207f736ca123f | e317aab93749d16d9ac58816cc5b53885f2cc68a |
refs/heads/master | <file_sep>using Microsoft.Extensions.DependencyInjection;
namespace ComponentsApp.UI.ViewModels
{
internal class ViewModelLocator
{
public MainWindowVm MainWindowVm => App.Host.Services.GetRequiredService<MainWindowVm>();
public ResultWindowVm ResultWindowVm => App.Host.Services.GetRequiredService<ResultWindowVm>();
}
}
<file_sep>using ComponentsApp.Data.Common;
using System.ComponentModel;
namespace ComponentsApp.Data.Models
{
public class Sample : BaseModel
{
#region Поля
private double _methane;
private double _ethane;
private double _propane;
private double _isobutane;
private double _butane;
private double _isopentane;
private double _pentane;
private double _hexane;
private double _nitrogen;
private double _oxygen;
private double _carbonDioxide;
#endregion
[DisplayName("Метан")]
public double Methane
{
get => _methane;
set => Set( ref _methane, value);
}
[DisplayName("Этан")]
public double Ethane
{
get => _ethane;
set => Set(ref _ethane, value);
}
[DisplayName("Пропан")]
public double Propane
{
get => _propane;
set => Set(ref _propane, value);
}
[DisplayName("и-Бутан")]
public double Isobutane
{
get => _isobutane;
set => Set(ref _isobutane, value);
}
[DisplayName("н-Бутан")]
public double Butane
{
get => _butane;
set => Set(ref _butane, value);
}
[DisplayName("и-Пентан")]
public double Isopentane
{
get => _isopentane;
set => Set(ref _isopentane, value);
}
[DisplayName("н-Пентан")]
public double Pentane
{
get => _pentane;
set => Set(ref _pentane, value);
}
[DisplayName("Гексан+высшие")]
public double Hexane
{
get => _hexane;
set => Set(ref _hexane, value);
}
[DisplayName("Азот")]
public double Nitrogen
{
get => _nitrogen;
set => Set(ref _nitrogen, value);
}
[DisplayName("Кислород")]
public double Oxygen
{
get => _oxygen;
set => Set(ref _oxygen, value);
}
[DisplayName("Диоксид углерода")]
public double CarbonDioxide
{
get => _carbonDioxide;
set => Set(ref _carbonDioxide, value);
}
public double Density { get; set; }
}
}
<file_sep>using ComponentsApp.Calculation.Interfaces;
using ComponentsApp.Data.Constants;
using ComponentsApp.Data.Models;
using System.Collections.Generic;
using System.Linq;
namespace ComponentsApp.Calculation.Services
{
public class CalculationService : ICalculationService
{
public Result Calculate(SamplePoint point1, SamplePoint point2, bool useDensity)
{
// Средние значения по каждой точке отбора
var avgSample1 = GetAvgSample(point1.Samples.ToHashSet());
var avgSample2 = GetAvgSample(point2.Samples.ToHashSet());
// Пересчитываем в массовые проценты
var massSample1 = GetMassSample(avgSample1);
var massSample2 = GetMassSample(avgSample2);
// Молярная масса смеси ПНГ, г/моль
var molarMass1 = GetMolarMass(avgSample1);
var molarMass2 = GetMolarMass(avgSample2);
// Расчет плотности при ст.у.
var density1 = useDensity ? avgSample1.Density : CalcDensity(molarMass1);
var density2 = useDensity ? avgSample2.Density : CalcDensity(molarMass2);
// Вычисление отобранной массы газа в точках отбора
var mass1 = point1.Volume * 1000 * density1;
var mass2 = point2.Volume * 1000 * density2;
// Соотношение массы ПНГ
var alpha = mass1 / (mass1 + mass2);
// Сумма компонентов C3+
var compSumm1 = GetComponentsSumm(massSample1);
var compSumm2 = GetComponentsSumm(massSample2);
// Средневзвешенная массовая концентрация фракций углеводородов
var wghtAvgConc = 10 * (alpha * density1 * compSumm1 + (1 - alpha) * density2 * compSumm2);
// Оформление результата
var result = new Result
{
SamplesCollection = new List<Sample> { massSample1, massSample2 },
MassCollection = new List<double> { molarMass1, molarMass2 },
DensityCollection = new List<double> { density1, density2 },
ComponentsSummCollection = new List<double> { compSumm1, compSumm2 },
WeightedAvgConc = wghtAvgConc
};
return result;
}
private double GetMolarMass(Sample sample)
{
return (sample.Methane * CompConstants.Methane +
sample.Ethane * CompConstants.Ethane +
sample.Propane * CompConstants.Propane +
sample.Isobutane * CompConstants.Isobutane +
sample.Butane * CompConstants.Butane +
sample.Isopentane * CompConstants.Isopentane +
sample.Pentane * CompConstants.Pentane +
sample.Hexane * CompConstants.Hexane +
sample.CarbonDioxide * CompConstants.CarbonDioxide +
sample.Oxygen * CompConstants.Oxigen +
sample.Nitrogen * CompConstants.Nitrogen) / 100;
}
private double CalcDensity(double molarMass) => molarMass / 23.9;
private Sample GetMassSample(Sample sample)
{
var result = new Sample();
var summary =
sample.Methane * CompConstants.Methane +
sample.Ethane * CompConstants.Ethane +
sample.Propane * CompConstants.Propane +
sample.Isobutane * CompConstants.Isobutane +
sample.Butane * CompConstants.Butane +
sample.Isopentane * CompConstants.Isopentane +
sample.Pentane * CompConstants.Pentane +
sample.Hexane * CompConstants.Hexane +
sample.CarbonDioxide * CompConstants.CarbonDioxide +
sample.Oxygen * CompConstants.Oxigen +
sample.Nitrogen * CompConstants.Nitrogen;
result.Methane = 100 * sample.Methane * CompConstants.Methane / summary;
result.Ethane = 100 * sample.Ethane * CompConstants.Ethane / summary;
result.Propane = 100 * sample.Propane * CompConstants.Propane / summary;
result.Isobutane = 100 * sample.Isobutane * CompConstants.Isobutane / summary;
result.Butane = 100 * sample.Butane * CompConstants.Butane / summary;
result.Isopentane = 100 * sample.Isopentane * CompConstants.Isopentane / summary;
result.Pentane = 100 * sample.Pentane * CompConstants.Pentane / summary;
result.Hexane = 100 * sample.Hexane * CompConstants.Hexane / summary;
result.CarbonDioxide = 100 * sample.CarbonDioxide * CompConstants.CarbonDioxide / summary;
result.Oxygen = 100 * sample.Oxygen * CompConstants.Oxigen / summary;
result.Nitrogen = 100 * sample.Nitrogen * CompConstants.Nitrogen / summary;
return result;
}
private Sample GetAvgSample(ICollection<Sample> samples)
{
return new Sample
{
Nitrogen = samples.Sum(s => s.Nitrogen) / samples.Count,
CarbonDioxide = samples.Sum(s => s.CarbonDioxide) / samples.Count,
Oxygen = samples.Sum(s => s.Oxygen) / samples.Count,
Methane = samples.Sum(s => s.Methane) / samples.Count,
Ethane = samples.Sum(s => s.Ethane) / samples.Count,
Propane = samples.Sum(s => s.Propane) / samples.Count,
Isobutane = samples.Sum(s => s.Isobutane) / samples.Count,
Butane = samples.Sum(s => s.Butane) / samples.Count,
Isopentane = samples.Sum(s => s.Isopentane) / samples.Count,
Pentane = samples.Sum(s => s.Pentane) / samples.Count,
Hexane = samples.Sum(s => s.Hexane) / samples.Count,
Density = samples.Sum(s => s.Density) / samples.Count,
};
}
private double GetComponentsSumm(Sample sample) =>
sample.Propane +
sample.Isobutane +
sample.Butane +
sample.Isopentane +
sample.Pentane +
sample.Hexane;
}
}
<file_sep>using MaterialDesignExtensions.Controls;
using System.Text.RegularExpressions;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Input;
namespace ComponentsApp.UI.Views
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : MaterialWindow
{
public MainWindow()
{
InitializeComponent();
}
private void mainTopPanel_MouseDown(object sender, MouseButtonEventArgs e)
{
DragMove();
}
private void Button_Click(object sender, RoutedEventArgs e)
{
var window = new AboutWindow();
window.ShowDialog();
}
private void TextBox_PreviewTextInput(object sender, TextCompositionEventArgs e)
{
var tb = (TextBox)sender;
Regex regex;
if (tb.Text.Contains("."))
{
if (tb.SelectedText.Length > 0 && tb.SelectedText.Contains("."))
{
regex = new Regex("[^0-9.]+");
}
else
{
regex = new Regex("[^0-9]+");
}
e.Handled = regex.IsMatch(e.Text);
}
else
{
regex = new Regex("[^0-9.]+");
e.Handled = regex.IsMatch(e.Text);
}
}
private void TextBox_KeyDown(object sender, KeyEventArgs e)
{
if (e.Key == Key.Enter)
{
var request = new TraversalRequest(FocusNavigationDirection.Next);
((UIElement)sender).MoveFocus(request);
var element = Keyboard.FocusedElement as TextBox;
if (element != null)
{
element.SelectAll();
}
}
}
}
}
<file_sep>using ComponentsApp.Data.Models;
namespace ComponentsApp.Calculation.Interfaces
{
public interface ICalculationService
{
Result Calculate(SamplePoint point1, SamplePoint point2, bool useDensity);
}
}<file_sep>using ComponentsApp.Data.Models;
using MigraDoc.DocumentObjectModel;
using MigraDoc.DocumentObjectModel.Tables;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
namespace ComponentsApp.Services.Services
{
public class PdfBuilderService
{
private static Document _document;
private static Result _data;
public static Document BuildDocument(Result data)
{
_data = data;
// Create a new MigraDoc document
_document = new Document();
_document.Info.Title = "Результаты расчета";
_document.Info.Subject = "";
_document.Info.Author = "ComponentsApp";
DefineStyles();
try
{
CreatePage();
}
catch (Exception e)
{
throw e;
}
//FillContent(data);
return _document;
}
private static void DefineStyles()
{
// Общий стиль документа
Style style = _document.Styles[StyleNames.Normal];
style.Font.Name = "Arial";
style.Font.Size = 12;
//style.ParagraphFormat.Font.Bold = true;
// Стиль заголовка
style = _document.Styles[StyleNames.Header];
style.ParagraphFormat.Alignment = ParagraphAlignment.Center;
style.ParagraphFormat.Font.Bold = true;
style.ParagraphFormat.Font.Size = 14;
style.ParagraphFormat.AddTabStop("10cm", TabAlignment.Center);
// Стиль подзаголовка
style = _document.Styles[StyleNames.Heading1];
style.ParagraphFormat.Alignment = ParagraphAlignment.Left;
style.ParagraphFormat.Font.Bold = true;
style.ParagraphFormat.Font.Size = 11;
style.ParagraphFormat.SpaceAfter = 10;
style.ParagraphFormat.SpaceBefore = 10;
// Стиль футтера
style = _document.Styles[StyleNames.Footer];
style.ParagraphFormat.AddTabStop("8cm", TabAlignment.Center);
// Стиль для табличных данных
style = _document.Styles.AddStyle("Table", StyleNames.Normal);
}
private static void CreatePage()
{
// Each MigraDoc document needs at least one section.
var section = _document.AddSection();
// Создаем футтер
var paragraph = section.Footers.Primary.AddParagraph();
paragraph.AddText($"Дата создания протокола: {DateTime.Now}");
paragraph.Format.Font.Size = 9;
paragraph.Format.Alignment = ParagraphAlignment.Center;
// Создаем заголовок страницы
paragraph = section.Headers.Primary.AddParagraph();
paragraph.AddText("Результат расчета средневзвешенной массовой концентрации фракций углеводородов С3+высшие в ПНГ");
paragraph.Style = StyleNames.Header;
// Создаем основной контент
// Создаем 1-й подзаголовок
paragraph = section.AddParagraph();
paragraph.AddText("1. Массовые доли компонентов ПНГ");
paragraph.Style = StyleNames.Heading1;
// Создаем таблицу "Массовые доли компонентов ПНГ"
var table = section.AddTable();
table.Format.Alignment = ParagraphAlignment.Center;
table.Rows.VerticalAlignment = VerticalAlignment.Center;
table.Rows.Height = 18;
table.Borders.Color = Colors.Black;
table.Borders.Width = 0.25;
table.Borders.Left.Width = 0.5;
table.Borders.Right.Width = 0.5;
table.Rows.LeftIndent = 0;
// Создаем столбцы таблицы
var column = table.AddColumn("2cm");
column.Format.Alignment = ParagraphAlignment.Left;
column = table.AddColumn("6cm");
column.Format.Alignment = ParagraphAlignment.Left;
table.AddColumn("4cm");
table.AddColumn("4cm");
// Создаем строки
var row = table.AddRow();
row.Cells[0].AddParagraph("№ п/п");
row.Cells[0].Format.Alignment = ParagraphAlignment.Center;
row.Cells[1].AddParagraph("Компонент");
row.Cells[1].Format.Alignment = ParagraphAlignment.Center;
row.Cells[2].AddParagraph("Массовая доля, %");
row.Cells[0].MergeDown = 1;
row.Cells[1].MergeDown = 1;
row.Cells[2].MergeRight = 1;
row = table.AddRow();
row.Cells[2].AddParagraph("1-ая группа МР");
row.Cells[3].AddParagraph("2-ая группа МР");
row = table.AddRow();
row.Format.Alignment = ParagraphAlignment.Center;
row.Cells[0].AddParagraph("1");
row.Cells[1].AddParagraph("2");
row.Cells[2].AddParagraph("3");
row.Cells[3].AddParagraph("4");
var properties = typeof(Sample).GetProperties();
for (int i = 0; i < properties.Length; i++)
{
if (properties[i].Name.Equals("Density")) break;
var name = properties[i]
.GetCustomAttributes(typeof(DisplayNameAttribute), true)
.Cast<DisplayNameAttribute>()
.First()
.DisplayName;
var value1 = (double)properties[i].GetValue(_data.SamplesCollection[0]);
var value2 = (double)properties[i].GetValue(_data.SamplesCollection[1]);
row = table.AddRow();
row.Cells[0].AddParagraph((i + 1).ToString());
row.Cells[1].AddParagraph(name);
row.Cells[2].AddParagraph(value1.ToString("f3"));
row.Cells[3].AddParagraph(value2.ToString("f3"));
}
table.SetEdge(0, 0, table.Columns.Count, table.Rows.Count, Edge.Box, BorderStyle.Single, 0.75);
// Создаем 2-й подзаголовок
paragraph = section.AddParagraph();
paragraph.AddText("2. Молярная масса ПНГ");
paragraph.Style = StyleNames.Heading1;
// Создаем таблицу "Молярная масса ПНГ"
table = section.AddTable();
table.Format.Alignment = ParagraphAlignment.Center;
table.Rows.VerticalAlignment = VerticalAlignment.Center;
table.Rows.Height = 18;
table.Borders.Color = Colors.Black;
table.Borders.Width = 0.25;
table.Borders.Left.Width = 0.5;
table.Borders.Right.Width = 0.5;
table.Rows.LeftIndent = 0;
// Создаем столбцы таблицы
table.AddColumn("8cm");
table.AddColumn("8cm");
// Создаем строки
row = table.AddRow();
row.Cells[0].AddParagraph("Молярная масса, г/моль");
row.Cells[0].MergeRight = 1;
row = table.AddRow();
row.Cells[0].AddParagraph("1-я группа МР");
row.Cells[1].AddParagraph("2-я группа МР");
row = table.AddRow();
row.Cells[0].AddParagraph(_data.MassCollection[0].ToString("f3"));
row.Cells[1].AddParagraph(_data.MassCollection[1].ToString("f3"));
table.SetEdge(0, 0, table.Columns.Count, table.Rows.Count, Edge.Box, BorderStyle.Single, 0.75);
// Создаем 3-й подзаголовок
paragraph = section.AddParagraph();
paragraph.AddText("3. Плотность ПНГ при стандарных условиях");
paragraph.Style = StyleNames.Heading1;
// Создаем таблицу "Плотность при стандартных условиях"
table = section.AddTable();
table.Format.Alignment = ParagraphAlignment.Center;
table.Rows.VerticalAlignment = VerticalAlignment.Center;
table.Rows.Height = 18;
table.Borders.Color = Colors.Black;
table.Borders.Width = 0.25;
table.Borders.Left.Width = 0.5;
table.Borders.Right.Width = 0.5;
table.Rows.LeftIndent = 0;
// Создаем столбцы таблицы
table.AddColumn("8cm");
table.AddColumn("8cm");
// Создаем строки
row = table.AddRow();
row.Cells[0].AddParagraph("Плотность при стандартных условиях, кг/м3");
row.Cells[0].MergeRight = 1;
row = table.AddRow();
row.Cells[0].AddParagraph("1-я группа МР");
row.Cells[1].AddParagraph("2-я группа МР");
row = table.AddRow();
row.Cells[0].AddParagraph(_data.DensityCollection[0].ToString("f5"));
row.Cells[1].AddParagraph(_data.DensityCollection[1].ToString("f5"));
table.SetEdge(0, 0, table.Columns.Count, table.Rows.Count, Edge.Box, BorderStyle.Single, 0.75);
// Создаем 4-й подзаголовок
paragraph = section.AddParagraph();
paragraph.AddText("4. Средневзвешенная массовая концентрация фракций углеводородов C3+высшие");
paragraph.Style = StyleNames.Heading1;
// Заполняем раздел
paragraph = section.AddParagraph();
paragraph.AddText($"Сумма массовых долей углеводородов C3+высшие первого МР равна {_data.ComponentsSummCollection[0]:f3}%");
paragraph.Style = StyleNames.Normal;
paragraph = section.AddParagraph();
paragraph.AddText($"Сумма массовых долей углеводородов C3+высшие второго МР равна {_data.ComponentsSummCollection[1]:f3}%");
paragraph.Style = StyleNames.Normal;
paragraph = section.AddParagraph();
paragraph.AddText($"Средневзвешенная массовая концентрация фракций углеводородов C3+высшие равна {_data.WeightedAvgConc:f2} г/моль");
paragraph.Style = StyleNames.Normal;
}
}
}
<file_sep>
using ComponentsApp.Calculation.Interfaces;
using ComponentsApp.Calculation.Services;
using ComponentsApp.Services.Interfaces;
using ComponentsApp.Services.Services;
using ComponentsApp.UI.ViewModels;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using System;
using System.Windows;
namespace ComponentsApp.UI
{
/// <summary>
/// Interaction logic for App.xaml
/// </summary>
public partial class App : Application
{
private static IHost _host;
public static IHost Host => _host ?? Program.CreateHostBuilder(Environment.GetCommandLineArgs()).Build();
public static void ConfigureServices(HostBuilderContext host, IServiceCollection services)
{
services.AddSingleton<ICalculationService, CalculationService>();
services.AddSingleton<IFileService, FileService>();
services.AddSingleton<MainWindowVm>();
services.AddSingleton<ResultWindowVm>();
}
protected override async void OnExit(ExitEventArgs e)
{
base.OnExit(e);
await Host.StopAsync().ConfigureAwait(false);
_host = null;
}
}
}
<file_sep>using MaterialDesignExtensions.Controls;
namespace ComponentsApp.UI.Views
{
/// <summary>
/// Логика взаимодействия для AboutWindow.xaml
/// </summary>
public partial class AboutWindow : MaterialWindow
{
public AboutWindow()
{
InitializeComponent();
}
}
}
<file_sep>namespace ComponentsApp.Data.Constants
{
public static class CompConstants
{
public const double Methane = 16.04246;
public const double Ethane = 30.06904;
public const double Propane = 44.09562;
public const double Isobutane = 58.1222;
public const double Butane = 58.1222;
public const double Isopentane = 72.14878;
public const double Pentane = 72.14878;
public const double Hexane = 86.17536;
public const double Nitrogen = 28.0134;
public const double Oxigen = 31.9988;
public const double CarbonDioxide = 44.0095;
}
}
<file_sep>using MaterialDesignExtensions.Controls;
namespace ComponentsApp.UI.Views
{
/// <summary>
/// Логика взаимодействия для ResultWindow.xaml
/// </summary>
public partial class ResultWindow : MaterialWindow
{
public ResultWindow()
{
InitializeComponent();
}
}
}
<file_sep>using ComponentsApp.Calculation.Interfaces;
using ComponentsApp.Data.Common;
using ComponentsApp.Data.Models;
using ComponentsApp.Services.Interfaces;
using ComponentsApp.UI.Infrastructure.Commands.Base;
using ComponentsApp.UI.Views;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Linq;
using System.Windows;
namespace ComponentsApp.UI.ViewModels
{
internal class MainWindowVm : BaseModel
{
#region Поля
private readonly IFileService _fileService;
private readonly ICalculationService _calculationService;
private bool _inputDensity;
private SamplePointVm _samplePoint1;
private SamplePointVm _samplePoint2;
private RelayCommand _closeApplicationCommand;
private RelayCommand _addSampleToSamplePoint1Command;
private RelayCommand _removeSampleFromSamplePoint1Command;
private RelayCommand _addSampleToSamplePoint2Command;
private RelayCommand _removeSampleFromSamplePoint2Command;
private RelayCommand _calculateCommand;
private RelayCommand _saveToFileCommand;
private RelayCommand _loadFromFileCommand;
#endregion
#region Свойства
// Вычислять плотность
public bool InputDensity
{
get => _inputDensity;
set => Set(ref _inputDensity, value);
}
// Коллекция проб с точки отбора №1
public SamplePointVm SamplePoint1
{
get => _samplePoint1;
set => Set(ref _samplePoint1, value);
}
// Коллекция проб с точки отбора №2
public SamplePointVm SamplePoint2
{
get => _samplePoint2;
set => Set(ref _samplePoint2, value);
}
private ResultWindowVm ResultWindowVm { get; }
#endregion
#region Комманды
// Закрыть приложение
public RelayCommand CloseApplicationCommand
{
get
{
if (_closeApplicationCommand == null)
{
_closeApplicationCommand = new RelayCommand(obj =>
{
Application.Current.Shutdown();
});
}
return _closeApplicationCommand;
}
}
// Добавить пробу для точки отбора #1
public RelayCommand AddSampleToSamplePoint1Command
{
get
{
if (_addSampleToSamplePoint1Command == null)
{
_addSampleToSamplePoint1Command = new RelayCommand(obj =>
{
var sampleNumber = SamplePoint1.Samples.Count + 1;
SamplePoint1.Samples.Add(new SampleVm { SampleNumber = sampleNumber });
},
c => SamplePoint1.Samples.Count != 10);
}
return _addSampleToSamplePoint1Command;
}
}
// Удалить пробу для точки отбора #1
public RelayCommand RemoveSampleFromSamplePoint1Command
{
get
{
if (_removeSampleFromSamplePoint1Command == null)
{
_removeSampleFromSamplePoint1Command = new RelayCommand(obj =>
{
var sample = SamplePoint1.Samples.Last();
SamplePoint1.Samples.Remove(sample);
},
c => SamplePoint1.Samples.Count > 1);
}
return _removeSampleFromSamplePoint1Command;
}
}
// Добавить пробу для точки отбора #2
public RelayCommand AddSampleToSamplePoint2Command
{
get
{
if (_addSampleToSamplePoint2Command == null)
{
_addSampleToSamplePoint2Command = new RelayCommand(obj =>
{
var sampleNumber = SamplePoint2.Samples.Count + 1;
SamplePoint2.Samples.Add(new SampleVm { SampleNumber = sampleNumber });
},
c => SamplePoint2.Samples.Count != 10);
}
return _addSampleToSamplePoint2Command;
}
}
// Удалить пробу для точки отбора #2
public RelayCommand RemoveSampleFromSamplePoint2Command
{
get
{
if (_removeSampleFromSamplePoint2Command == null)
{
_removeSampleFromSamplePoint2Command = new RelayCommand(obj =>
{
var sample = SamplePoint2.Samples.Last();
SamplePoint2.Samples.Remove(sample);
},
c => SamplePoint2.Samples.Count > 1);
}
return _removeSampleFromSamplePoint2Command;
}
}
// Расчитать результат
public RelayCommand CalculateCommand
{
get
{
if (_calculateCommand == null)
{
_calculateCommand = new RelayCommand(obj =>
{
if (SamplePoint1.Volume == 0)
{
MessageBox.Show("Объем газа для точки отбора №1 не может быть 0.", "Ошибка", MessageBoxButton.OK, MessageBoxImage.Warning);
}
else if (SamplePoint2.Volume == 0)
{
MessageBox.Show("Объем газа для точки отбора №2 не может быть 0.", "Ошибка", MessageBoxButton.OK, MessageBoxImage.Warning);
}
else if (!SamplePoint1.Samples.All(s => s.Summ == 100.0m))
{
MessageBox.Show("Сумма компонентов для каждой пробы в точке отбора №1 должна быть 100%.", "Ошибка", MessageBoxButton.OK, MessageBoxImage.Warning);
}
else if (!SamplePoint2.Samples.All(s => s.Summ == 100.0m))
{
MessageBox.Show("Сумма компонентов для каждой пробы в точке отбора №2 должна быть 100%.", "Ошибка", MessageBoxButton.OK, MessageBoxImage.Warning);
}
else
{
if (InputDensity)
{
if (SamplePoint1.Samples.Any(s => s.Sample.Density == 0) ||
SamplePoint2.Samples.Any(s => s.Sample.Density == 0))
{
MessageBox.Show("Плотность каждой из проб должна быть больше 0.", "Ошибка", MessageBoxButton.OK, MessageBoxImage.Warning);
return;
}
}
var samplePoint1 = new SamplePoint
{
Samples = SamplePoint1.Samples.Select(s => s.Sample).ToHashSet(),
Volume = SamplePoint1.Volume
};
var samplePoint2 = new SamplePoint
{
Samples = SamplePoint2.Samples.Select(s => s.Sample).ToHashSet(),
Volume = SamplePoint2.Volume
};
ResultWindowVm.Result = _calculationService.Calculate(samplePoint1, samplePoint2, InputDensity);
var resultWindow = new ResultWindow { DataContext = ResultWindowVm };
resultWindow.ShowDialog();
}
});
}
return _calculateCommand;
}
}
public RelayCommand SaveToFileCommand
{
get
{
if (_saveToFileCommand == null)
{
_saveToFileCommand = new RelayCommand(async obj =>
{
var data = new List<SamplePoint>
{
new SamplePoint{ Samples = SamplePoint1.Samples.Select(s=> s.Sample).ToHashSet(), Volume = SamplePoint1.Volume },
new SamplePoint{ Samples = SamplePoint2.Samples.Select(s=> s.Sample).ToHashSet(), Volume = SamplePoint2.Volume },
};
var result = await _fileService.SaveDataAsync(data);
if (result)
{
MessageBox.Show("Данные успешно сохранены", "Сохранение данных", MessageBoxButton.OK, MessageBoxImage.Information);
}
else
{
MessageBox.Show("Ошибка сохранения", "Сохранение данных", MessageBoxButton.OK, MessageBoxImage.Error);
}
});
}
return _saveToFileCommand;
}
}
public RelayCommand LoadFromFileCommand
{
get
{
if (_loadFromFileCommand == null)
{
_loadFromFileCommand = new RelayCommand(async obj =>
{
var result = (await _fileService.LoadDataAsync())?.ToArray();
if (result != null)
{
var samples1 = new List<SampleVm>();
foreach (var sample in result[0].Samples)
{
samples1.Add(new SampleVm { Sample = sample, SampleNumber = samples1.Count + 1 });
}
var samples2 = new List<SampleVm>();
foreach (var sample in result[1].Samples)
{
samples2.Add(new SampleVm { Sample = sample, SampleNumber = samples2.Count + 1 });
}
SamplePoint1.Volume = result[0].Volume;
SamplePoint1.Samples = new ObservableCollection<SampleVm>(samples1);
SamplePoint2.Volume = result[1].Volume;
SamplePoint2.Samples = new ObservableCollection<SampleVm>(samples2);
}
else
{
MessageBox.Show("Нет сохраненных данных", "Загрузка данных", MessageBoxButton.OK, MessageBoxImage.Information);
}
});
}
return _loadFromFileCommand;
}
}
#endregion
public MainWindowVm(
ResultWindowVm resultWindowVm,
IFileService fileService,
ICalculationService calculationService)
{
ResultWindowVm = resultWindowVm;
_fileService = fileService;
_calculationService = calculationService;
SamplePoint1 = new SamplePointVm
{
Header = "ВГПП ПНГ Сепарация УВКС Е-1/1,2",
SubHeader = "Варьеганское, Тагринское и Новоаганское месторождения"
};
SamplePoint2 = new SamplePointVm
{
Header = "ВГПП ПНГ Сепарация Узел №1",
SubHeader = "Рославльское и Западно-Варьеганское месторождения"
};
SamplePoint1.Samples.Add(new SampleVm { SampleNumber = 1 });
SamplePoint1.Samples.Add(new SampleVm { SampleNumber = 2 });
SamplePoint1.Samples.Add(new SampleVm { SampleNumber = 3 });
SamplePoint1.Samples.Add(new SampleVm { SampleNumber = 4 });
SamplePoint2.Samples.Add(new SampleVm { SampleNumber = 1 });
SamplePoint2.Samples.Add(new SampleVm { SampleNumber = 2 });
SamplePoint2.Samples.Add(new SampleVm { SampleNumber = 3 });
SamplePoint2.Samples.Add(new SampleVm { SampleNumber = 4 });
}
}
}
<file_sep>using ComponentsApp.Data.Models;
using ComponentsApp.Services.Interfaces;
using MigraDoc.Rendering;
using System;
using System.Collections.Generic;
using System.IO;
using System.Text;
using System.Text.Json;
using System.Threading.Tasks;
namespace ComponentsApp.Services.Services
{
public class FileService : IFileService
{
private readonly JsonSerializerOptions _options;
public FileService()
{
_options = new JsonSerializerOptions { WriteIndented = true };
}
public async Task<bool> SaveDataAsync(IEnumerable<SamplePoint> samplePoints)
{
var data = JsonSerializer.Serialize(samplePoints, _options);
try
{
await File.WriteAllTextAsync("SavedData.json", data);
return true;
}
catch (Exception)
{
return false;
}
}
public async Task<IEnumerable<SamplePoint>> LoadDataAsync()
{
try
{
var data = await File.ReadAllTextAsync("SavedData.json");
return JsonSerializer.Deserialize<IEnumerable<SamplePoint>>(data);
}
catch
{
return null;
}
}
public void SaveToPdf(Result data, string filePath)
{
var document = PdfBuilderService.BuildDocument(data);
Encoding.RegisterProvider(CodePagesEncodingProvider.Instance);
var pdfRenderer = new PdfDocumentRenderer(true);
pdfRenderer.Document = document;
pdfRenderer.RenderDocument();
pdfRenderer.PdfDocument.Save(filePath);
}
}
}
<file_sep>using System.Collections.Generic;
namespace ComponentsApp.Data.Models
{
public class SamplePoint
{
public ICollection<Sample> Samples { get; set; }
public double Volume { get; set; }
}
}
<file_sep>using ComponentsApp.Data.Models;
using System.Collections.Generic;
using System.Threading.Tasks;
namespace ComponentsApp.Services.Interfaces
{
public interface IFileService
{
void SaveToPdf(Result data, string filePath);
Task<bool> SaveDataAsync(IEnumerable<SamplePoint> samplePoints);
Task<IEnumerable<SamplePoint>> LoadDataAsync();
}
}
<file_sep>using ComponentsApp.Data.Common;
using System.Collections.ObjectModel;
namespace ComponentsApp.UI.ViewModels
{
public class SamplePointVm : BaseModel
{
private ObservableCollection<SampleVm> _samples;
private double _volume;
public string Header { get; set; }
public string SubHeader { get; set; }
public double Volume
{
get => _volume;
set => Set(ref _volume, value);
}
public ObservableCollection<SampleVm> Samples
{
get => _samples;
set => Set(ref _samples, value);
}
public SamplePointVm()
{
Samples = new ObservableCollection<SampleVm>();
}
}
}
<file_sep>using System.Collections.Generic;
namespace ComponentsApp.Data.Models
{
public class Result
{
public IList<Sample> SamplesCollection { get; set; }
public IList<double> MassCollection { get; set; }
public IList<double> DensityCollection { get; set; }
public IList<double> ComponentsSummCollection { get; set; }
public double WeightedAvgConc { get; set; }
}
}
<file_sep>using ComponentsApp.Data.Common;
using ComponentsApp.Data.Models;
using System.ComponentModel;
namespace ComponentsApp.UI.ViewModels
{
public class SampleVm : BaseModel
{
private Sample _sample;
public int SampleNumber { get; set; }
public Sample Sample
{
get => _sample;
set
{
Set(ref _sample, value);
_sample.PropertyChanged += SamplePropertyChanged;
}
}
public decimal Summ => (decimal)
(Sample.Methane
+ Sample.Ethane
+ Sample.Propane
+ Sample.Isobutane
+ Sample.Butane
+ Sample.Isopentane
+ Sample.Pentane
+ Sample.Hexane
+ Sample.Nitrogen
+ Sample.Oxygen
+ Sample.CarbonDioxide);
public SampleVm()
{
Sample = new Sample();
Sample.PropertyChanged += SamplePropertyChanged;
}
private void SamplePropertyChanged(object sender, PropertyChangedEventArgs e)
{
OnPropertyChanged(nameof(Summ));
}
}
}
<file_sep>using ComponentsApp.Data.Common;
using ComponentsApp.Data.Models;
using ComponentsApp.Services.Interfaces;
using ComponentsApp.UI.Infrastructure.Commands.Base;
using Microsoft.Win32;
using System;
namespace ComponentsApp.UI.ViewModels
{
internal class ResultWindowVm : BaseModel
{
public ResultWindowVm(IFileService fileService)
{
_fileService = fileService;
}
#region Поля
private readonly IFileService _fileService;
private RelayCommand _saveToPdfCommand;
#endregion
#region Свойства
public Result Result { get; set; }
#endregion
#region Комманды
public RelayCommand SaveToPdfCommand
{
get
{
if (_saveToPdfCommand == null)
{
_saveToPdfCommand = new RelayCommand(obj =>
{
var sfd = new SaveFileDialog
{
Filter = "Pdf Document|*.pdf",
Title = "Save an PDF File",
FileName = $"Протокол расчета {DateTime.Now.ToShortDateString()}.pdf"
};
if (sfd.ShowDialog() == true)
{
_fileService.SaveToPdf(Result, sfd.FileName);
}
});
}
return _saveToPdfCommand;
}
}
#endregion
}
}
| ab55a68f89e24fd3a7b77ca220558c3d6552bf43 | [
"C#"
] | 18 | C# | Odis25/ComponentsApp | 93edae2ebab0b8db8ed5b75387bf32403df58c0e | 2baa92027ff7cb4db116bcb731b7c6d27f72a1ee |
refs/heads/master | <repo_name>ghada-dhif/React-Native<file_sep>/src/informationPersonelles/index.js
/* eslint-disable prettier/prettier */
import InfoPerScreen from './InformationPersonelles';
export {InfoPerScreen};
<file_sep>/src/creationEmployee/CreationEmployee.js
/* eslint-disable prettier/prettier */
import React, {Component, useState} from 'react';
import {
View,
Text,
Button,
Image,
Picker,
StyleSheet,
SafeAreaView,
ScrollView,
TouchableHighlight,
Alert,
TouchableOpacity,
} from 'react-native';
import {TextInput} from 'react-native-gesture-handler';
//import database from '@react-native-firebase/database';
import Modal from 'react-native-modal';
export default class CreationScreen extends Component {
state = {user: ''};
updateUser = (user) => {
this.setState({user: user});
};
state = {
isModalVisible: false,
isModal2Visible: false,
};
toggleModal = () => {
this.setState({
isModalVisible: !this.state.isModalVisible,
});
};
toggleModal2 = () => {
this.setState({
isModal2Visible: !this.state.isModal2Visible,
});
};
render() {
return (
<SafeAreaView>
<ScrollView>
<View style={styles.container}>
<View style={{flexDirection: 'row'}}>
<TouchableOpacity style={styles.Btn} onPress={this.toggleModal}>
<Text style={{color: 'white'}}>Informations Générales</Text>
</TouchableOpacity>
<TouchableOpacity style={styles.Btn} onPress={this.toggleModal2}>
<Text style={{color: 'white'}}>Informations Personelles</Text>
</TouchableOpacity>
</View>
<View>
<Modal isVisible={this.state.isModalVisible}>
<View style={styles.modalView}>
<Text style={styles.logo}>Hello!</Text>
<Text style={styles.logoA}>Entrez vos informations!</Text>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Nom De l'employé: "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Poste Occupé: "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handlePoste}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={8}
underlineColorAndroid="transparent"
placeholder="télephone:"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleTelephone}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Adresse:"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleAdresse}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Lieu de Travail: "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Gestionnaire: "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<View style={{flexDirection: 'row'}}>
<Text>Départements: </Text>
<Picker
selectedValue={this.state.user}
onValueChange={this.updateUser}
style={{height: 50, width: 150}}>
<Picker.Item label="" value="" />
<Picker.Item label="Informatique" value="Informatique" />
<Picker.Item label="Mecanique" value="Mecanique" />
<Picker.Item label="Electrique" value="Electrique" />
</Picker>
</View>
<View style={{flexDirection: 'row'}}>
<TouchableOpacity style={styles.Btn}>
<Text style={{color: 'white'}}>Comfirmer</Text>
</TouchableOpacity>
<TouchableOpacity
style={styles.Btn}
onPress={this.toggleModal}>
<Text style={{color: 'white'}}>Annuler</Text>
</TouchableOpacity>
</View>
</View>
</Modal>
<Modal isVisible={this.state.isModal2Visible}>
<View style={styles.modalView}>
<Text>hello</Text>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Adresse:"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={16}
underlineColorAndroid="transparent"
placeholder="Numéro de Compte Bancaire:"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={4}
underlineColorAndroid="transparent"
placeholder="Km Domicile - Bureau:"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={8}
underlineColorAndroid="transparent"
placeholder="Contact d'urgence:"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={8}
underlineColorAndroid="transparent"
placeholder="Téléphone d'urgence:"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Champ d’étude:"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="École:"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<View style={{flexDirection: 'row'}}>
<Text
style={{
textAlign: 'left',
fontWeight: 'bold',
borderRadius: 1,
}}>
Etat Civil:
</Text>
<Picker
style={{borderWidth: 1}}
selectedValue={this.state.user}
onValueChange={this.updateUser}>
<Picker.Item label="" value="" />
<Picker.Item label="célibtaires" value="célibtaires" />
<Picker.Item label="Marié" value="Marié" />
<Picker.Item label="Dévorsé(e)" value="Dévorsé(e)" />
</Picker>
</View>
<View style={{flexDirection: 'row'}}>
<Text style={{textAlign: 'left', fontWeight: 'bold'}}>
Niveau De Certificat:
</Text>
<Picker
selectedValue={this.state.selectedValue}
onValueChange={(itemValue, itemIndex) =>
this.setState({selectedValue: itemValue})
}>
<Picker.Item label="" value="" />
<Picker.Item label="Bachelier" value="Bachelier" />
<Picker.Item label="Master" value="Master" />
</Picker>
</View>
<View style={{flexDirection: 'row'}}>
<TouchableOpacity style={styles.Btn}>
<Text style={{color: 'white'}}>Comfirmer</Text>
</TouchableOpacity>
<TouchableOpacity
style={styles.Btn}
onPress={this.toggleModal2}>
<Text style={{color: 'white'}}>Annuler</Text>
</TouchableOpacity>
</View>
</View>
</Modal>
</View>
</View>
</ScrollView>
</SafeAreaView>
);
}
}
const styles = StyleSheet.create({
centeredView: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
marginTop: 22,
},
openButton: {
backgroundColor: '#F194FF',
borderRadius: 20,
padding: 10,
elevation: 2,
},
Btn: {
width: '50%',
backgroundColor: '#fb5b5a',
borderRadius: 8,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 15,
marginBottom: 10,
},
logo: {
fontWeight: 'bold',
fontSize: 40,
color: '#fb5b5a',
marginBottom: 10,
alignItems: 'center',
},
logoA: {
fontWeight: 'bold',
fontSize: 20,
color: '#fb5b5a',
marginBottom: 10,
alignItems: 'center',
},
modalView: {
margin: 20,
backgroundColor: 'white',
borderRadius: 20,
padding: 35,
alignItems: 'center',
shadowColor: '#000',
shadowOffset: {
width: 0,
height: 2,
},
shadowOpacity: 0.25,
shadowRadius: 3.84,
elevation: 5,
},
textStyle: {
color: 'white',
fontWeight: 'bold',
textAlign: 'center',
},
modalText: {
marginBottom: 15,
textAlign: 'center',
},
inputView: {
width: '100%',
borderWidth: 1,
borderColor: 'black',
borderRadius: 8,
fontSize: 15,
fontWeight: 'bold',
alignSelf: 'center',
},
});
<file_sep>/src/achats/Achats.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View, Text, Button, StyleSheet, TouchableOpacity, SafeAreaView, ScrollView} from 'react-native';
import {Container, Header, Left, Body, Right, Title, Item, Input, Icon} from 'native-base';
import ActionButton from 'react-native-action-button';
//import { TouchableOpacity } from 'react-native-gesture-handler';
export default class AchatScreen extends Component {
constructor(props) {
super(props);
}
render() {
console.log(this.props);
return (
<SafeAreaView>
<ScrollView>
<Container>
<Header>
<Right>
<TouchableOpacity
onPress={() => {
this.props.navigation.toggleDrawer();
}}>
<Icon
name="menu"
/>
</TouchableOpacity>
</Right>
</Header>
<View style={styles.container}>
<Text style={styles.logo}>Achats Screen</Text>
<ActionButton
buttonColor="rgba(231,76,60,1)"
onPress={() => this.props.navigation.navigate('CreationAchat')}
/>
</View>
</Container>
</ScrollView>
</SafeAreaView>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#003f5c',
alignItems: 'center',
justifyContent: 'center',
},
inputView: {
width: '80%',
backgroundColor: '#465881',
borderRadius: 25,
height: 50,
marginBottom: 20,
justifyContent: 'center',
padding: 20,
},
inputText: {
height: 50,
color: 'white',
},
forgot: {
color: 'white',
fontSize: 11,
},
loginBtn: {
width: '80%',
backgroundColor: '#fb5b5a',
borderRadius: 25,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 40,
marginBottom: 10,
},
logo: {
fontWeight: 'bold',
fontSize: 40,
color: '#fb5b5a',
marginBottom: 50,
alignItems: 'center',
},
});<file_sep>/src/contacts/Contacts.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {
View,
Text,
StyleSheet,
SafeAreaView,
ScrollView,
TouchableOpacity,
TextInput,
FlatList,
} from 'react-native';
import {createBottomTabNavigator} from '@react-navigation/bottom-tabs';
import {CreationContactScreen} from '../creationContact';
import FontAwesome5 from 'react-native-vector-icons/FontAwesome5';
import ActionButton from 'react-native-action-button';
import Modal from 'react-native-modal';
//import database from '@react-native-firebase/database';
export default class ContactsScreen extends Component {
constructor(props) {
super(props);
}
state = {
isModalVisible: false,
isModal2Visible: false,
isModal3Visible: false,
nom: '',
societe: '',
rue: '',
Rue2: '',
TVA: '',
Poste: '',
telephone: '',
mobile: '',
web: '',
etat: '',
ville: '',
code: '',
};
toggleModal = () => {
this.setState({
isModalVisible: !this.state.isModalVisible,
});
};
toggleModal2 = () => {
this.setState({
isModal2Visible: !this.state.isModal2Visible,
});
};
toggleModal3 = () => {
this.setState({
isModal3Visible: !this.state.isModal3Visible,
});
};
updateUser = (user) => {
this.setState({user: user});
};
handleNom = (text) => {
this.setState({nom: text});
};
handleRue = (text) => {
this.setState({rue: text});
};
handleRue2 = (text) => {
this.setState({rue2: text});
};
handleTVA = (text) => {
this.setState({TVA: text});
};
handlePoste = (text) => {
this.setState({Poste: text});
};
handleTelephone = (text) => {
this.setState({telephone: text});
};
handleMobile = (text) => {
this.setState({mobile: text});
};
handleWeb = (text) => {
this.setState({web: text});
};
handleVille = (text) => {
this.setState({ville: text});
};
handleEtat = (text) => {
this.setState({etat: text});
};
handleCode = (text) => {
this.setState({code: text});
};
handleSociete = (text) => {
this.setState({societe: text});
};
/***********function db
writeuserdata = () => {
database()
.ref('/contacts/' + this.state.nom + this.state.societe)
.update({
nom: this.state.nom,
rue: this.state.rue,
rue2: this.state.rue2,
societe: this.state.societe,
TVA: this.state.TVA,
Poste: this.state.Poste,
telephone: this.state.telephone,
web: this.state.web,
ville: this.state.Ville,
etat: this.state.etat,
code: this.state.code,
})
.then((data) => {
console.log('data', data);
})
.catch((error) => {
console.log('error', error);
});
};
/**fetching data
fetchData = async () => {
let array = [];
this.setState({
loading: true,
});
database()
.ref('contacts/')
.on('value', (snapshot) => {
snapshot.forEach((element) => {
array.push(element._snapshot.value);
});
this.setState({
data: array,
loading: false,
});
});
};
componentDidMount() {
this.fetchData();
}*/
render() {
console.log(this.state.data);
return (
<View style={styles.container}>
<Text style={styles.logo}>Contacts Screen</Text>
<FlatList
refreshing={this.state.loading}
data={this.state.data}
renderItem={({item}) => (
<TouchableOpacity style={styles.contactsItem}>
<Text style={styles.text}>nom :{item.nom}</Text>
<Text>rue: {item.rue}</Text>
<Text>rue2: {item.rue2}</Text>
<Text>societe: {item.societe}</Text>
<Text>TVA: {item.TVA}</Text>
<Text>Poste: {item.Poste}</Text>
<Text>telephone: {item.telephone}</Text>
<Text>web: {item.web}</Text>
<Text>ville: {item.ville}</Text>
<Text>etat: {item.etat}</Text>
<Text>code: {item.code}</Text>
</TouchableOpacity>
)}
ItemSeparatorComponent={() => {
return (
<View
style={{
borderBottomWidth: 0.5,
borderBottomColor: 'red',
}}
/>
);
}}
keyExtractor={(item) => item.key}
/>
<ActionButton
buttonColor="rgba(231,76,60,1)"
onPress={this.toggleModal}
/>
<Modal isVisible={this.state.isModalVisible}>
<View style={styles.modalView}>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Nom "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleNom}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Societé: "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleSociete}
/>
<Text>Adresse de la société</Text>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Rue.. "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleRue}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Rue2.. "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleRue}
/>
<View style={{flexDirection: 'row'}}>
<TextInput
style={styles.inputView1}
underlineColorAndroid="transparent"
placeholder="Ville "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleVille}
/>
<TextInput
style={styles.inputView1}
underlineColorAndroid="transparent"
placeholder="Etat "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEtat}
/>
<TextInput
style={styles.inputView1}
underlineColorAndroid="transparent"
placeholder="Code Postal "
keyboardType="numeric"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleCode}
/>
</View>
<TextInput
style={styles.inputView2}
underlineColorAndroid="transparent"
placeholder="N:TVA:e.g.BE0477472701 "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleTVA}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Poste Occupée "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handlePoste}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Téléphone "
keyboardType="numeric"
maxLength={8}
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleTelephone}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Mobile "
keyboardType="numeric"
maxLength={8}
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleMobile}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Lien vers un site web "
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleWeb}
/>
<View style={{flexDirection: 'row'}}>
<TouchableOpacity style={styles.Btn}
onPress={() => this.writeuserdata()}>
<Text style={{color: 'white'}}>Comfirmer</Text>
</TouchableOpacity>
<TouchableOpacity style={styles.Btn} onPress={this.toggleModal}>
<Text style={{color: 'white'}}>Annuler</Text>
</TouchableOpacity>
</View>
</View>
</Modal>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#003f5c',
//alignItems: 'center',
justifyContent: 'center',
},
text: {
color: 'red',
fontSize: 11,
},
Btn: {
width: '50%',
backgroundColor: '#fb5b5a',
borderRadius: 8,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 15,
marginBottom: 10,
margin: 5,
},
modalText: {
marginBottom: 15,
textAlign: 'center',
},
inputView: {
width: '100%',
borderWidth: 1,
borderColor: 'black',
borderRadius: 8,
fontSize: 15,
fontWeight: 'bold',
alignSelf: 'center',
margin: 2,
},
inputView1: {
width: '40%',
borderWidth: 1,
borderColor: 'black',
borderRadius: 8,
fontSize: 15,
fontWeight: 'bold',
alignSelf: 'center',
margin: 2,
},
inputView2: {
width: '100%',
borderWidth: 1,
borderColor: 'black',
borderRadius: 8,
fontSize: 15,
fontWeight: 'bold',
alignSelf: 'center',
margin: 2,
},
inputText: {
height: 50,
color: 'white',
},
forgot: {
color: 'white',
fontSize: 11,
},
loginBtn: {
width: '80%',
backgroundColor: '#fb5b5a',
borderRadius: 25,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 40,
marginBottom: 10,
},
logo: {
fontWeight: 'bold',
fontSize: 30,
color: '#fb5b5a',
marginBottom: 50,
alignItems: 'center',
},
modalView: {
margin: 10,
backgroundColor: 'white',
borderRadius: 20,
padding: 35,
alignItems: 'center',
shadowColor: '#000',
shadowOffset: {
width: 0,
height: 2,
},
shadowOpacity: 0.25,
shadowRadius: 3.84,
elevation: 5,
},
contactsItem: {
backgroundColor: 'white',
margin: 5,
width: '70%',
borderRadius: 6,
padding: 50,
marginTop: 15,
elevation: 5,
alignSelf:'center',
},
item: {
backgroundColor: '#f9c2ff',
padding: 20,
marginVertical: 8,
marginHorizontal: 16,
},
title: {
fontSize: 32,
},
});
<file_sep>/src/login/index.js
/* eslint-disable prettier/prettier */
import LoginScreen from './src/login';
export {LoginScreen};
<file_sep>/src/donnee/index.js
/* eslint-disable prettier/prettier */
import DonneeScreen from './donnee';
export {DonneeScreen};
<file_sep>/src/congNav/index.js
/* eslint-disable prettier/prettier */
import CongNav from './CongNav';
export {CongNav};
<file_sep>/src/methode/methode.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {
View,
TouchableOpacity,
Text,
StyleSheet,
SafeAreaView,
ScrollView,
} from 'react-native';
import {CheckBox} from 'react-native-elements';
import SelectMultiple from 'react-native-select-multiple';
//import { TouchableOpacity } from 'react-native-gesture-handler';
const fruits = [
'Signature en ligne',
'PayPal',
'Carte de crédit (via Stripe)',
'Autre intermédiaire de Paiement',
'Instructions de paiement',
];
export default class MethodeScreen extends Component {
state = {selectedFruits: []};
onSelectionsChange = (selectedFruits) => {
// selectedFruits is array of { label, value }
this.setState({selectedFruits});
};
render() {
return (
<SafeAreaView>
<ScrollView>
<View style={styles.container}>
<Text style={styles.logo}>methode de payement </Text>
</View>
<View>
<SelectMultiple
items={fruits}
selectedItems={this.state.selectedFruits}
onSelectionsChange={this.onSelectionsChange}
/>
</View>
</ScrollView>
</SafeAreaView>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#003f5c',
alignItems: 'center',
justifyContent: 'center',
},
inputView: {
width: '80%',
backgroundColor: '#465881',
borderRadius: 25,
height: 50,
marginBottom: 20,
justifyContent: 'center',
padding: 20,
},
inputText: {
height: 50,
color: 'white',
},
forgot: {
color: 'white',
fontSize: 11,
},
loginBtn: {
width: '80%',
backgroundColor: '#fb5b5a',
borderRadius: 25,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 40,
marginBottom: 10,
},
logo: {
fontWeight: 'bold',
fontSize: 30,
color: '#fb5b5a',
marginBottom: 50,
alignItems: 'center',
},
});
<file_sep>/src/myDrawer/index.js
/* eslint-disable prettier/prettier */
import DrawerScreen from './myDrawer';
export {DrawerScreen};
<file_sep>/src/creationContact/index.js
/* eslint-disable prettier/prettier */
import CreationContactScreen from './CreationContact';
export {CreationContactScreen};
<file_sep>/src/header/index.js
/* eslint-disable prettier/prettier */
import HeaderScreen from './Header';
export {HeaderScreen};
<file_sep>/src/myDrawer/Drawer.js
/* eslint-disable react-native/no-inline-styles */
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {NavigationContainer} from '@react-navigation/native';
import {
createDrawerNavigator,
} from '@react-navigation/drawer';
import ContactsScreen from '../contacts/Contacts';
import TabScreen from '../tab/Tab';
import {CongesScreen} from '../conges';
import {CongNav} from '../congNav';
import {CrmScreen} from '../crm';
import {ServiceScreen} from '../service';
import {MapsScreen} from '../maps';
import {PannScreen} from '../pann';
import {SettingScreen} from '../setting';
import NavigationScreen from '../navigation/Navigation';
import HeaderScreen from '../header/Header';
import VenteNavigator from '../venteNavigator/VenteNavigator';
import DrawerContents from '../drawerContents';
import {View, Image, Icon, Content} from 'native-base';
import FontAwesome5 from 'react-native-vector-icons/FontAwesome5';
import {
Text,
TouchableOpacity,
} from 'react-native';
import AchatNavigator from '../achatNavigator/AchatNavigator';
import {StyleSheet, ScrollView} from 'react-native';
import {Caption, Title, Avatar} from 'react-native-paper';
import {DrawerContentScrollView} from '@react-navigation/drawer';
import {EmployeesScreen} from '../employees';
import {AboutScreen} from '../about';
//import {LandingView} from '../landingView';
function DrawerContent(props) {
return (
<DrawerContentScrollView {...props}>
<View style={styles.container}>
<View style={{height: 150}}>
<Avatar.Image source={require('../img/ghada.jpg')} />
<Title><NAME></Title>
<Caption>@Ghada1edhif</Caption>
</View>
<View style={{backgroundColor: 'white', height: 5}} />
<View style={{height: 600}}>
<View style={{flexDirection: 'row'}}>
<TouchableOpacity
{...props}
style={styles.loginBtn}
onPress={() => props.navigation.navigate('Feed')}>
<Icon name="" />
<Text style={{color: 'black'}}>Acceuil</Text>
</TouchableOpacity>
</View>
<TouchableOpacity
{...props}
style={styles.loginBtn}
onPress={() => props.navigation.navigate('pann')}>
<Icon name="" />
<Text style={{color: 'black'}}>pann</Text>
</TouchableOpacity>
<TouchableOpacity
{...props}
style={styles.loginBtn}
onPress={() => props.navigation.navigate('service')}>
<Icon name="" />
<Text style={{color: 'black'}}>Service Sur Site</Text>
</TouchableOpacity>
<TouchableOpacity
{...props}
style={styles.loginBtn}
onPress={() => props.navigation.navigate('employees')}>
<Icon name="" />
<Text style={{color: 'black'}}>Employés</Text>
</TouchableOpacity>
<TouchableOpacity
{...props}
style={styles.loginBtn}
onPress={() => props.navigation.navigate('vente')}>
<Icon name="" />
<Text style={{color: 'black'}}>Ventes</Text>
</TouchableOpacity>
<TouchableOpacity
{...props}
style={styles.loginBtn}
onPress={() => props.navigation.navigate('crm')}>
<Text style={{color: 'black'}}>Crm</Text>
</TouchableOpacity>
<TouchableOpacity
{...props}
style={styles.loginBtn}
onPress={() => props.navigation.navigate('contacts')}>
<Icon name="" />
<Text style={{color: 'black'}}>Contacts</Text>
</TouchableOpacity>
<TouchableOpacity
{...props}
style={styles.loginBtn}
onPress={() => props.navigation.navigate('Achats')}>
<Icon name="" />
<Text style={{color: 'black'}}>Achats</Text>
</TouchableOpacity>
<TouchableOpacity
{...props}
style={styles.loginBtn}
onPress={() => props.navigation.navigate('Conges')}>
<Text style={{color: 'black'}}>Congés</Text>
</TouchableOpacity>
<TouchableOpacity
{...props}
style={styles.loginBtn}
onPress={() => props.navigation.navigate('maps')}>
<Icon name="" />
<Text style={{color: 'black'}}>maps</Text>
</TouchableOpacity>
</View>
</View>
<View style={{backgroundColor: 'white', height: 5}} />
<View style={styles.containerA}>
<TouchableOpacity
style={styles.Btn}
{...props}
onPress={() => props.navigation.navigate('About')}>
<Text style={{color: 'black'}}>About Us</Text>
</TouchableOpacity>
<TouchableOpacity
{...props}
onPress={() => props.navigation.navigate('LandingView')}
style={styles.Btn}>
<Text style={{color: 'black'}}>LogOut</Text>
</TouchableOpacity>
</View>
</DrawerContentScrollView>
);
}
const DrawerNav = createDrawerNavigator();
// Important NOTE : SET THE DRAWER TO CONTAIN BOTTOM TAB
class DrawerScreen extends Component {
constructor(props) {
super(props);
}
render() {
return (
<DrawerNav.Navigator
// eslint-disable-next-line react-native/no-inline-styles
drawerStyle={{
width: 320,
}}
drawerType={'slide'}
initialRouteName="tabsHome"
drawerContent={() => <DrawerContent {...this.props} />}>
<DrawerNav.Screen name="tabsHome" component={TabScreen} />
<DrawerNav.Screen name="employees" component={NavigationScreen} />
<DrawerNav.Screen name="congés" component={CongesScreen} />
<DrawerNav.Screen name="vente" component={VenteNavigator} />
<DrawerNav.Screen name="About" component={AboutScreen} />
<DrawerNav.Screen name="maps" component={MapsScreen} />
<DrawerNav.Screen name="crm" component={CrmScreen} />
<DrawerNav.Screen name="contacts" component={ContactsScreen} />
<DrawerNav.Screen name="service" component={ServiceScreen} />
<DrawerNav.Screen name="pann" component={PannScreen} />
</DrawerNav.Navigator>
);
}
}
export default DrawerScreen;
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#003f5c',
alignItems: 'stretch',
justifyContent: 'center',
},
containerA: {
flex: 1,
backgroundColor: '#003f5c',
alignItems: 'stretch',
justifyContent: 'center',
flexDirection: 'row',
},
inputView: {
width: '80%',
backgroundColor: '#465881',
borderRadius: 25,
height: 50,
marginBottom: 20,
justifyContent: 'center',
padding: 20,
},
inputText: {
height: 50,
color: 'white',
},
forgot: {
color: 'white',
fontSize: 11,
},
loginBtn: {
width: '80%',
backgroundColor: '#fb5b5a',
borderRadius: 25,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 15,
marginBottom: 10,
flexDirection: 'row',
},
logo: {
fontWeight: 'bold',
fontSize: 40,
color: '#fb5b5a',
marginBottom: 50,
alignItems: 'center',
},
Btn: {
width: '30%',
backgroundColor: '#fb5b5a',
borderRadius: 25,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 15,
marginBottom: 10,
margin: 0.5,
},
});
<file_sep>/src/landingContainer/landingContainer.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View} from 'react-native';
import {createStackNavigator} from '@react-navigation/stack';
import {NavigationContainer} from '@react-navigation/native';
import LandingView from '../landingView/landingView';
import DrawerScreen from '../myDrawer/Drawer';
const Stack = createStackNavigator();
export default class LandingContainer extends Component {
render() {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen
name="landing"
component={LandingView}
options={{
headerShown: false,
}}
/>
<Stack.Screen
name={'mainDrawer'}
component={DrawerScreen}
options={{
headerShown: false,
}}
/>
</Stack.Navigator>
</NavigationContainer>
);
}
}
<file_sep>/src/creationVente/CreationVente.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {
View,
Text,
StyleSheet,
ScrollView,
SafeAreaView,
TouchableOpacity,
TextInput,
} from 'react-native';
import Modal from 'react-native-modal';
import SelectMultiple from 'react-native-select-multiple';
const fruits = [
'Signature en ligne',
'PayPal',
'Carte de crédit (via Stripe)',
'Autre intermédiaire de Paiement',
'Instructions de paiement',
];
export default class CreationVenteScreen extends Component {
state = {user: ''};
updateUser = (user) => {
this.setState({user: user});
};
state = {
isModalVisible: false,
isModal2Visible: false,
};
toggleModal = () => {
this.setState({
isModalVisible: !this.state.isModalVisible,
});
};
toggleModal2 = () => {
this.setState({
isModal2Visible: !this.state.isModal2Visible,
});
};
state = {selectedFruits: []};
onSelectionsChange = (selectedFruits) => {
// selectedFruits is array of { label, value }
this.setState({selectedFruits});
};
render() {
return (
<SafeAreaView>
<ScrollView>
<View style={styles.container}>
<View style={{flexDirection: 'row'}}>
<TouchableOpacity style={styles.Btn} onPress={this.toggleModal}>
<Text style={{color: 'white'}}>Données Sur La Societé</Text>
</TouchableOpacity>
<TouchableOpacity style={styles.Btn} onPress={this.toggleModal2}>
<Text style={{color: 'white'}}>Methode De Payement</Text>
</TouchableOpacity>
</View>
<View>
<Modal isVisible={this.state.isModalVisible}>
<View style={styles.modalView}>
<Text style={styles.logo}>Hello!</Text>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={24}
underlineColorAndroid="transparent"
placeholder="Nom de la société"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<Text style={styles.logoA}>Adresse:</Text>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={24}
underlineColorAndroid="transparent"
placeholder="RUE"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={24}
underlineColorAndroid="transparent"
placeholder="RUE2"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
maxLength={24}
underlineColorAndroid="transparent"
placeholder="Ville"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={24}
underlineColorAndroid="transparent"
placeholder="Code Postale"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={24}
underlineColorAndroid="transparent"
placeholder="Telephone"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={24}
underlineColorAndroid="transparent"
placeholder="courriel"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
maxLength={24}
underlineColorAndroid="transparent"
placeholder="Site Web"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={24}
underlineColorAndroid="transparent"
placeholder="Numéro de TVA"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={24}
underlineColorAndroid="transparent"
placeholder="Registre De lA Société"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<View style={{flexDirection: 'row'}}>
<TouchableOpacity style={styles.Btn}>
<Text style={{color: 'white'}}>Comfirmer</Text>
</TouchableOpacity>
<TouchableOpacity
style={styles.Btn}
onPress={this.toggleModal}>
<Text style={{color: 'white'}}>Annuler</Text>
</TouchableOpacity>
</View>
</View>
</Modal>
<Modal isVisible={this.state.isModal2Visible}>
<View style={styles.modalView}>
<Text>hello</Text>
<SelectMultiple
items={fruits}
selectedItems={this.state.selectedFruits}
onSelectionsChange={this.onSelectionsChange}
/>
<View style={{flexDirection: 'row'}}>
<TouchableOpacity style={styles.Btn}>
<Text style={{color: 'white'}}>Comfirmer</Text>
</TouchableOpacity>
<TouchableOpacity
style={styles.Btn}
onPress={this.toggleModal2}>
<Text style={{color: 'white'}}>Annuler</Text>
</TouchableOpacity>
</View>
</View>
</Modal>
</View>
</View>
</ScrollView>
</SafeAreaView>
);
}
}
const styles = StyleSheet.create({
centeredView: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
marginTop: 22,
},
openButton: {
backgroundColor: '#F194FF',
borderRadius: 20,
padding: 10,
elevation: 2,
},
Btn: {
width: '50%',
backgroundColor: '#fb5b5a',
borderRadius: 8,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 15,
marginBottom: 10,
},
logo: {
fontWeight: 'bold',
fontSize: 40,
color: '#fb5b5a',
marginBottom: 10,
alignItems: 'center',
},
logoA: {
fontWeight: 'bold',
fontSize: 20,
color: '#fb5b5a',
marginBottom: 10,
alignItems: 'center',
},
modalView: {
margin: 20,
backgroundColor: 'white',
borderRadius: 20,
padding: 35,
alignItems: 'center',
shadowColor: '#000',
shadowOffset: {
width: 0,
height: 2,
},
shadowOpacity: 0.25,
shadowRadius: 3.84,
elevation: 5,
},
textStyle: {
color: 'white',
fontWeight: 'bold',
textAlign: 'center',
},
modalText: {
marginBottom: 15,
textAlign: 'center',
},
inputView: {
width: '100%',
borderWidth: 1,
borderColor: 'black',
borderRadius: 8,
fontSize: 15,
fontWeight: 'bold',
alignSelf: 'center',
},
});
<file_sep>/src/setting/index.js
/* eslint-disable prettier/prettier */
import SettingScreen from './setting';
export {SettingScreen};
<file_sep>/src/about/About.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {
View,
StyleSheet,
ScrollView,
SafeAreaView,
Linking,
} from 'react-native';
import {CardItem, Card, Text, Body, } from 'native-base';
import FontAwesome5 from 'react-native-vector-icons/FontAwesome5';
export default class AboutScreen extends Component {
render() {
return (
<SafeAreaView>
<ScrollView>
<View style={styles.container}>
<Text style={styles.logo}>About us Screen</Text>
</View>
<View style={{backgroundColor: '#fb5b5a', height: 5}} />
<View style={styles.containerA}>
<Text style={styles.logoA}>
CONTACTER - ALPHA ENGINEERING INTERNATIONAL:
</Text>
</View>
<View>
<Text>SAHLOUL 3</Text>
<Text> Université de Sousse</Text>
<Text>4054 Sousse</Text>
<Text>Fax +216 73 821 249</Text>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL('http://www.alpha-engineering.com.tn/')
}>
Alpha Engineering
</Text>
</View>
<Card>
<CardItem header bordered>
<Text>
INFORMATIONS JURIDIQUE - ALPHA ENGINEERING INTERNATIONAL
</Text>
</CardItem>
<CardItem bordered>
<Body>
<Text>Année de création ==> 2006</Text>
<Text>
Forme juridique ==> Société A Responsabilité limitée (S.A.R.L.)
</Text>
<Text>Kompass ID ==> TN106187</Text>
</Body>
</CardItem>
</Card>
<Card>
<CardItem header bordered>
<Text>
PRÉSENTATION - ALPHA ENGINEERING INTERNATIONAL
</Text>
</CardItem>
<CardItem bordered>
<Body>
<Text>
Une société d'ingénierie leader établi en 2004 en offrant à ses
clients une gamme complète de services d'ingénierie, de gestion, de
supervision et de formation dans l'industrie du pétrole et du gaz:{' '}
</Text>
<Text>- Oil & Gas Projets Ingénierie</Text>
<Text>- Oil & Gas packages pré-ingénierie </Text>
<Text>- Gestion de projet Oil & Gas </Text>
<Text>- Oil & Gas Project Control</Text>
<Text>- Oil & Gas formation </Text>
<Text>- supervision Oil & Gas champ</Text>
</Body>
</CardItem>
</Card>
<Card>
<CardItem header bordered>
<Text >
ACTIVITÉS PRINCIPALES SELON LA CLASSIFICATION KOMPASS
</Text>
</CardItem>
<CardItem bordered>
<Body>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL(
'https://tn.kompass.com/a/conseil-en-ingenierie-pour-l-extraction-et-l-industrie-du-petrole/84380/',
)
}>
*Conseil en ingénierie pour l'extraction et l'industrie du pétrole
et du gaz
</Text>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL(
'https://tn.kompass.com/a/conseil-en-prospection-de-petrole-et-de-gaz/8438001/',
)
}>
** Conseil en prospection de pétrole et de gaz
</Text>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL(
'https://tn.kompass.com/a/conseil-en-extraction-de-petrole-et-de-gaz/8438002/',
)
}>
*** Conseil en extraction de pétrole et de gaz
</Text>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL(
'https://tn.kompass.com/a/etude-de-projets-pour-ingenierie-de-recherche-petroliere/8438003/',
)
}>
***Etude de projets pour ingénierie de recherche pétrolière
</Text>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL(
'https://tn.kompass.com/a/conseil-en-ingenierie-pour-l-extraction-petroliere-en-mer/8438005/',
)
}>
*** Conseil en ingénierie pour l'extraction pétrolière en mer
</Text>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL(
'https://tn.kompass.com/a/conseil-en-injection-d-eau-pour-puits-de-petrole/8438006/',
)
}>
***Conseil en injection d'eau pour puits de pétrole
</Text>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL(
'https://tn.kompass.com/a/conseil-en-ingenierie-pour-l-inspection-de-plates-formes-petrolieres/8438007/',
)
}>
***Conseil en ingénierie pour l'inspection de plates-formes
pétrolières
</Text>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL(
'https://tn.kompass.com/a/conseil-en-installation-et-deplacement-de-plates-formes-petrolieres/8438008/',
)
}>
***Conseil en installation et déplacement de plates-formes
pétrolières
</Text>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL(
'https://tn.kompass.com/a/services-pour-cables-de-forage-de-puits-de-petrole/8438009/',
)
}>
***Services pour câbles de forage de puits de pétrole
</Text>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL(
'https://tn.kompass.com/a/conseil-en-ingenierie-pour-l-inspection-de-plates-formes-petrolieres/8438007/',
)
}>
***Conseil en ingénierie pour l'inspection de plates-formes
pétrolières
</Text>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL(
'https://tn.kompass.com/a/conseil-en-installation-et-deplacement-de-plates-formes-petrolieres/8438008/',
)
}>
***Conseil en installation et déplacement de plates-formes
pétrolières
</Text>
<Text
style={{color: 'blue'}}
onPress={() =>
Linking.openURL(
'https://tn.kompass.com/a/services-pour-cables-de-forage-de-puits-de-petrole/8438009/',
)
}>
***Services pour câbles de forage de puits de pétrole
</Text>
</Body>
</CardItem>
</Card>
</ScrollView>
</SafeAreaView>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#003f5c',
alignItems: 'center',
justifyContent: 'center',
},
containerA: {
flex: 1,
backgroundColor: '#003f5c',
alignItems: 'center',
justifyContent: 'center',
marginBottom: 20,
},
containerB: {
flex: 1,
backgroundColor: 'white',
alignItems: 'center',
justifyContent: 'center',
marginBottom: 20,
},
inputView: {
width: '90%',
borderWidth: 1,
borderColor: 'black',
borderRadius: 25,
fontSize: 15,
fontWeight: 'bold',
alignSelf: 'center',
},
inputText: {
height: 50,
color: 'white',
},
forgot: {
color: 'white',
fontSize: 11,
},
loginBtn: {
width: '80%',
backgroundColor: '#fb5b5a',
borderRadius: 25,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 40,
marginBottom: 10,
},
logo: {
fontWeight: 'bold',
fontSize: 40,
color: '#fb5b5a',
marginBottom: 50,
alignItems: 'center',
},
logoA: {
fontWeight: 'bold',
fontSize: 20,
color: 'white',
marginBottom: 10,
alignItems: 'center',
},
Btn: {
width: '100%',
backgroundColor: '#fb5b5a',
borderRadius: 25,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 15,
marginBottom: 10,
},
});
<file_sep>/src/creationAchat/CreationAchat.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View, Text, Button, SafeAreaView, TextInputMask, Picker, StyleSheet, Modal} from 'react-native';
import {TextInput, ScrollView, TouchableOpacity} from 'react-native-gesture-handler';
import DatePicker from 'react-native-datepicker';
export default class CreationAchatScreen extends Component {
state = {user: ''};
updateUser = (user) => {
this.setState({user: user});
}
constructor(props) {
super(props);
//set value in state for initial date
this.state = {date: '15-05-2018'};
}
render() {
return (
<SafeAreaView >
<ScrollView>
<View
style={styles.container}>
<Text style={styles.logo}>
Créer Achats{' '}
</Text>
<Text style={styles.logo} >Demande de Prix</Text>
</View>
<View style={{backgroundColor: '#fb5b5a', height: 5}} />
<View style={styles.containerA} >
<Text style={styles.logoA}>Fournisseur:</Text>
</View>
<View style={styles.containerB} >
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={24}
underlineColorAndroid="transparent"
placeholder="Nom,nméro de TVA, courriel ou référance"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={8}
underlineColorAndroid="transparent"
placeholder="Référance Fournisseur:"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
</View>
<View style={styles.containerB} />
<View style={styles.containerA} >
<Text style={styles.logoA}>Date De La Commande:</Text>
</View>
<View style={styles.containerB} >
<DatePicker
style={{width: 200}}
date={this.state.date} //initial date from state
mode="date" //The enum of date, datetime and time
placeholder="select date"
format="DD-MM-YYYY"
minDate="01-01-2016"
maxDate="01-01-2030"
confirmBtnText="Confirm"
cancelBtnText="Cancel"
customStyles={{
dateIcon: {
position: 'absolute',
left: 0,
top: 4,
marginLeft: 0,
},
dateInput: {
marginLeft: 36,
},
}}
onDateChange={(date) => {
this.setState({date: date});
}}
/>
</View>
<View style={styles.containerA} >
<Text style={styles.logoA}>Article: </Text>
</View>
<View >
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Article"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Description Article"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
</View>
<View >
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={8}
underlineColorAndroid="transparent"
placeholder="Quantité: 0.000"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
underlineColorAndroid="transparent"
placeholder="Prix Unitaire:0.000"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
</View>
<View >
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={8}
underlineColorAndroid="transparent"
placeholder="Taxe"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<TextInput
style={styles.inputView}
keyboardType="numeric"
maxLength={8}
underlineColorAndroid="transparent"
placeholder="Sous Total:
0.000"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
<View style={styles.containerA} >
<Text style={styles.logoA}>Autres Informations: </Text>
</View>
<View >
<Text style={{Color: 'black'}}>Dates De Reception:</Text>
<DatePicker
style={{width: 200}}
date={this.state.date}
mode="date"
placeholder="select date"
format="DD-MM-YYYY"
minDate="01-05-2016"
maxDate="01-05-2030"
confirmBtnText="Confirm"
cancelBtnText="Cancel"
customStyles={{
dateIcon: {
position: 'absolute',
left: 0,
top: 4,
marginLeft: 0,
},
dateInput: {
marginLeft: 36,
},
// ... You can check the source to find the other keys.
}}
onDateChange={(date) => {this.setState({date: date});}}
/>
<TextInput
style={styles.inputView}
underlineColorAndroid="transparent"
placeholder="Responcable Achat"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
</View>
<View style={{backgroundColor: 'white', height: 5}} />
<View style={styles.containerA}>
<Text style={styles.logoA}>
Conditions de paiement:
</Text>
</View>
<View>
<Picker
style={{borderWidth: 1}}
selectedValue={this.state.user}
onValueChange={this.updateUser}>
<Picker.Item label="" value="" />
<Picker.Item label="Paiement Immédiat" value="Paiement Immédiat" />
<Picker.Item label="15 jours" value="15 jours" />
<Picker.Item label="21 jours" value="21 jours" />
<Picker.Item label="30 jours" value="30 jours" />
<Picker.Item label=" Fin De Mois" value="Fin De Mois" />
</Picker>
</View>
<View style={styles.containerA}>
<Text style={styles.logoA}>Position Fiscale</Text>
</View>
<TextInput
style={{
width: '90%',
borderWidth: 1,
borderColor: 'black',
borderRadius: 25,
fontSize: 15,
fontWeight: 'bold',
alignSelf: 'center',
}}
underlineColorAndroid="transparent"
placeholder="Position Fiscale"
placeholderTextColor="grey"
autoCapitalize="none"
onChangeText={this.handleEmployee}
/>
</View >
<View style={styles.containerA}>
<View style={{flexDirection: 'row'}} >
<TouchableOpacity
style={styles.Btn}>
<Text style={{color: 'white'}}>Comfirmer</Text>
</TouchableOpacity>
<TouchableOpacity
style={styles.Btn}>
<Text style={{color: 'white'}}>Annuler</Text>
</TouchableOpacity>
</View>
</View>
</ScrollView>
</SafeAreaView>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#003f5c',
alignItems: 'center',
justifyContent: 'center',
},
containerA: {
flex: 1,
backgroundColor: '#003f5c',
alignItems: 'center',
justifyContent: 'center',
marginBottom: 20,
},
containerB: {
flex: 1,
backgroundColor: 'white',
alignItems: 'center',
justifyContent: 'center',
marginBottom: 20,
},
inputView: {
width: '90%',
borderWidth: 1,
borderColor: 'black',
borderRadius: 25,
fontSize: 15,
fontWeight: 'bold',
alignSelf: 'center',
},
inputText: {
height: 50,
color: 'white',
},
forgot: {
color: 'white',
fontSize: 11,
},
loginBtn: {
width: '80%',
backgroundColor: '#fb5b5a',
borderRadius: 25,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 40,
marginBottom: 10,
},
logo: {
fontWeight: 'bold',
fontSize: 40,
color: '#fb5b5a',
marginBottom: 50,
alignItems: 'center',
},
logoA: {
fontWeight: 'bold',
fontSize: 20,
color: 'white',
marginBottom: 10,
alignItems: 'center',
},
Btn: {
width: '100%',
backgroundColor: '#fb5b5a',
borderRadius: 25,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 15,
marginBottom: 10,
},
});
<file_sep>/src/about/index.js
/* eslint-disable prettier/prettier */
import AboutScreen from './About';
export {AboutScreen};
<file_sep>/src/creationVenteNav/creationVenteNav.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View, Text, Button} from 'react-native';
import {createStackNavigator} from '@react-navigation/stack';
import {CreationVenteScreen} from '../creationVente';
import {DonneeScreen} from '../donnee';
import {MethodeScreen} from '../methode';
import {MiseScreen} from '../mise';
const Stack = createStackNavigator();
export default class CreationVenteNavigator extends Component {
render() {
return (
<Stack.Navigator>
<Stack.Screen name="CreationVente" component={CreationVenteScreen} />
<Stack.Screen name="donnee" component={DonneeScreen} />
<Stack.Screen name="mise" component={MiseScreen} />
<Stack.Screen name="methode" component={MethodeScreen} />
</Stack.Navigator>
);
}
}
<file_sep>/src/mapsNav/index.js
/* eslint-disable prettier/prettier */
import mapsNav from './maps';
export {mapsNav};
<file_sep>/src/maps/maps.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {
View,
Text,
Button,
StyleSheet,
TouchableOpacity,
SafeAreaView,
ScrollView,
} from 'react-native';
import MapView from 'react-native-maps';
import {Marker} from 'react-native-maps';
import Permissions from 'react-native-maps';
//import { TouchableOpacity } from 'react-native-gesture-handler';
export default class MapsScreen extends Component {
render() {
return (
<MapView
style={{flex: 1, height: 400, width: 400}}
initialRegion={{
latitude: 35.764252,
longitude: 10.811289,
latitudeDelta: 0.0922,
longitudeDelta: 0.0421,
}}></MapView>
);
}
}
<file_sep>/src/service/index.js
/* eslint-disable prettier/prettier */
import ServiceScreen from './service';
export {ServiceScreen};
<file_sep>/src/contactNavigation/contactNavigation.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View, Text, Button} from 'react-native';
import {createStackNavigator} from '@react-navigation/stack';
import {CreationContactScreen} from '../creationContact';
import {ContactsScreen} from '../contacts';
const Stack = createStackNavigator();
export default class ContactNavigator extends Component {
render() {
return (
<Stack.Navigator>
<Stack.Screen name="Contact" component={ContactsScreen} />
<Stack.Screen name="CreationContact" component={CreationContactScreen} />
</Stack.Navigator>
);
}
}
<file_sep>/src/venteNavigator/VenteNavigator.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View, Text, Button} from 'react-native';
import {createStackNavigator} from '@react-navigation/stack';
import {VenteScreen} from '../ventes';
import {CreationVenteNavigator} from '../creationVenteNav';
import {CreationVenteScreen} from '../creationVente';
const Stack = createStackNavigator();
export default class VenteNavigator extends Component {
render() {
return (
<Stack.Navigator>
<Stack.Screen name="Vente" component={VenteScreen} />
<Stack.Screen name="CreationVente" component={CreationVenteNavigator} />
</Stack.Navigator>
);
}
}
<file_sep>/src/accNavigation/AccNavigation.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View, Text, Button} from 'react-native';
import {createStackNavigator} from '@react-navigation/stack';
import {FeedScreen} from '../feed';
import DrawerScreen from '../myDrawer/Drawer';
const Stack = createStackNavigator();
export default class AccNavigator extends Component {
render() {
return (
<Stack.Navigator>
<Stack.Screen name="Feed" component={FeedScreen} />
<Stack.Screen name="mainDrawer" component={DrawerScreen} />
</Stack.Navigator>
);
}
}
<file_sep>/src/mise/index.js
/* eslint-disable prettier/prettier */
import MiseScreen from './mise';
export {MiseScreen};
<file_sep>/App.js
import React, {Component} from 'react';
import DrawerScreen from './src/myDrawer/Drawer';
import LandingContainer from './src/landingContainer/landingContainer';
export default class App extends Component {
render() {
return <LandingContainer />;
}
}
<file_sep>/src/employees/index.js
/* eslint-disable prettier/prettier */
import EmployeesScreen from './Employees';
export {EmployeesScreen};
<file_sep>/src/informations professionelles/index.js
/* eslint-disable prettier/prettier */
import InfoProScreen from './InfoPro';
export {InfoProScreen};
<file_sep>/src/navigation/index.js
/* eslint-disable prettier/prettier */
import NavigationScreen from './Navigation';
export {NavigationScreen};
<file_sep>/src/mapsNav/maps.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View, Text, Button} from 'react-native';
import {createStackNavigator} from '@react-navigation/stack';
import {CongesScreen} from '../conges';
import MapsScreen from '../maps/maps';
import DrawerScreen from '../myDrawer/Drawer';
import {NavigationContainer} from '@react-navigation/native';
const Stack = createStackNavigator();
export default class mapsNav extends Component {
render() {
return (
<NavigationContainer initialRouteName="mainDrawer">
<Stack.Navigator>
<Stack.Screen name="maps" component={MapsScreen} />
<Stack.Screen name="mainDrawer" component={DrawerScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
}
<file_sep>/src/accNavigation/index.js
/* eslint-disable prettier/prettier */
import AccNavigationScreen from './AccNavigation';
export {AccNavigationScreen};
<file_sep>/src/header/Header.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View, Text, Button, Header} from 'react-native';
export default class HeaderScreen extends Component{
render() {
return (
<Header
leftComponent={{ icon: 'menu', color: '#red' }}
centerComponent={{ text: 'MY TITLE', style: { color: '#fff' } }}
rightComponent={{ icon: 'home', color: '#fff' }}
/>
);
}
}
<file_sep>/src/creationVenteNav/index.js
/* eslint-disable prettier/prettier */
import CreationVenteNavigator from './creationVenteNav';
export {CreationVenteNavigator};
<file_sep>/src/creationAchat/index.js
/* eslint-disable prettier/prettier */
import CreationAchatScreen from './CreationAchat';
export {CreationAchatScreen};
<file_sep>/src/navigation/Navigation.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View, Text, Button, LogoTitle} from 'react-native';
import {createStackNavigator} from '@react-navigation/stack';
import {EmployeesScreen} from '../employees';
import CreationScreen from '../creationEmployee/CreationEmployee';
import {FeedScreen} from '../feed';
import {CrmScreen} from '../crm';
import {InfoPerScreen} from '../informationPersonelles';
import {InfoProScreen} from '../informations professionelles';
import {CreationAchatScreen} from '../creationAchat';
import {AchatsScreen} from '../achats';
import AchatNavigator from '../achatNavigator/AchatNavigator';
import { CongNav } from '../congNav';
const Stack = createStackNavigator();
export default class NavigationScreen extends Component {
render() {
return (
// eslint-disable-next-line react-native/no-inline-styles
<Stack.Navigator initialRouteName="Employees">
<Stack.Screen
name="Employees"
options={{headerShown: false}}
component={EmployeesScreen}
/>
<Stack.Screen name="Creation" component={CreationScreen} />
<Stack.Screen name="Feed" component={FeedScreen} />
<Stack.Screen name="InfoPer" component={InfoPerScreen} />
<Stack.Screen name="InfoPro" component={InfoProScreen} />
<Stack.Screen name="Achat" component={AchatNavigator} />
<Stack.Screen name="Conges" component={CongNav} />
</Stack.Navigator>
);
}
}
<file_sep>/src/methode/index.js
/* eslint-disable prettier/prettier */
import MethodeScreen from './methode';
export {MethodeScreen};
<file_sep>/src/conges/index.js
/* eslint-disable prettier/prettier */
import CongesScreen from './Conges';
export {CongesScreen};
<file_sep>/src/ventes/index.js
/* eslint-disable prettier/prettier */
import VenteScreen from './Ventes';
export {VenteScreen};
<file_sep>/src/mise/mise.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View, Text, Button, StyleSheet, TouchableOpacity, SafeAreaView, ScrollView} from 'react-native';
import {Container, Header, Left, Body, Right, Title, Item, Input, Icon} from 'native-base';
//import { TouchableOpacity } from 'react-native-gesture-handler';
export default class MiseScreen extends Component {
render() {
return (
<View style={styles.container}>
<Text style={styles.logo}>mise Screen</Text>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#003f5c',
alignItems: 'center',
justifyContent: 'center',
},
inputView: {
width: '80%',
backgroundColor: '#465881',
borderRadius: 25,
height: 50,
marginBottom: 20,
justifyContent: 'center',
padding: 20,
},
inputText: {
height: 50,
color: 'white',
},
forgot: {
color: 'white',
fontSize: 11,
},
loginBtn: {
width: '80%',
backgroundColor: '#fb5b5a',
borderRadius: 25,
height: 50,
alignItems: 'center',
justifyContent: 'center',
marginTop: 40,
marginBottom: 10,
},
logo: {
fontWeight: 'bold',
fontSize: 40,
color: '#fb5b5a',
marginBottom: 50,
alignItems: 'center',
},
});
<file_sep>/src/creationVente/index.js
/* eslint-disable prettier/prettier */
import CreationVenteScreen from './CreationVente';
export {CreationVenteScreen};
<file_sep>/src/feed/index.js
/* eslint-disable prettier/prettier */
import FeedScreen from './Feed';
export {FeedScreen};
<file_sep>/src/achatNavigator/AchatNavigator.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View, Text, Button} from 'react-native';
import {createStackNavigator} from '@react-navigation/stack';
import {AchatsScreen} from '../achats';
import {CreationAchatScreen} from '../creationAchat';
const Stack = createStackNavigator();
export default class AchatNavigator extends Component {
render() {
return (
<Stack.Navigator>
<Stack.Screen name="Achat" component={AchatsScreen} />
<Stack.Screen name="CreationAchat" component={CreationAchatScreen} />
</Stack.Navigator>
);
}
}
<file_sep>/src/contacts/index.js
/* eslint-disable prettier/prettier */
import ContactsScreen from './Contacts';
export {ContactsScreen};
<file_sep>/src/maps/index.js
/* eslint-disable prettier/prettier */
import MapsScreen from './maps';
export {MapsScreen};
<file_sep>/src/creationContact/CreationContact.js
/* eslint-disable prettier/prettier */
import React, {Component} from 'react';
import {View, Text, StyleSheet, SafeAreaView, ScrollView} from 'react-native';
import {createBottomTabNavigator} from '@react-navigation/bottom-tabs';
export default class CreationContactScreen extends Component {
render() {
return (
<SafeAreaView>
<ScrollView>
<View>
<Text> creation Contacts Screen</Text>
</View>
<View style={{backgroundColor: '#fb5b5a', height: 5}} />
</ScrollView>
</SafeAreaView>
);
}
}
| 91eaf3e21bd8cbf65bb69564ebeeee6de71fc4b5 | [
"JavaScript"
] | 46 | JavaScript | ghada-dhif/React-Native | 2e8bbab7bb3689cb7bd13c219fe4e69e5322ae08 | 50fb017b8f8bc4abe682fbfa0ef20f27fc3d3887 |
refs/heads/master | <repo_name>zig-for/AginahBot<file_sep>/bot.js
const { Client, Collection } = require('discord.js')
const config = require('./config.json');
const {generalErrorHandler} = require('./errorHandlers');
const { verifyModeratorRole, verifyIsAdmin, handleGuildCreate, handleGuildDelete,
verifyGuildSetups, cachePartial } = require('./lib');
const fs = require('fs');
// Catch all unhandled errors
process.on('uncaughtException', (err) => generalErrorHandler(err));
const client = new Client({ partials: [ 'GUILD_MEMBER', 'MESSAGE', 'REACTION' ] });
client.devMode = process.argv[2] && process.argv[2] === 'dev';
client.commands = new Collection();
client.commandCategories = [];
client.messageListeners = [];
client.reactionListeners = [];
client.voiceStateListeners = [];
// Load command category files
fs.readdirSync('./commandCategories').filter((file) => file.endsWith('.js')).forEach((categoryFile) => {
const commandCategory = require(`./commandCategories/${categoryFile}`);
client.commandCategories.push(commandCategory);
commandCategory.commands.forEach((command) => {
client.commands.set(command.name, command);
});
});
// Load message listener files
fs.readdirSync('./messageListeners').filter((file) => file.endsWith('.js')).forEach((listenerFile) => {
const listener = require(`./messageListeners/${listenerFile}`);
client.messageListeners.push(listener);
});
// Load reaction listener files
fs.readdirSync('./reactionListeners').filter((file) => file.endsWith('.js')).forEach((listenerFile) => {
const listener = require(`./reactionListeners/${listenerFile}`);
client.reactionListeners.push(listener);
});
// Load voice state listener files
fs.readdirSync('./voiceStateListeners').filter((file) => file.endsWith('.js')).forEach((listenerFile) => {
const listener = require(`./voiceStateListeners/${listenerFile}`);
client.voiceStateListeners.push(listener);
});
client.on('message', async(message) => {
// Fetch message if partial
message = await cachePartial(message);
if (message.member) { message.member = await cachePartial(message.member); }
if (message.author) { message.author = await cachePartial(message.author); }
// Ignore all bot messages
if (message.author.bot) { return; }
// If the message does not begin with the command prefix, run it through the message listeners
if (!message.content.startsWith(config.commandPrefix)) {
return client.messageListeners.forEach((listener) => listener(client, message));
}
// If the message is a command, parse the command and arguments
// TODO: Allow arguments to be enclosed in single or double quotes
const args = message.content.slice(config.commandPrefix.length).trim().split(/ +/);
const commandName = args.shift().toLowerCase();
try{
// Get the command object
const command = client.commands.get(commandName) ||
client.commands.find((cmd) => cmd.aliases && cmd.aliases.includes(commandName));
// If the command does not exist, alert the user
if (!command) { return message.channel.send("I don't know that command. Use `!aginah help` for more info."); }
// If the command does not require a guild, just run it
if (!command.guildOnly) { return command.execute(message, args); }
// If this message was not sent from a guild, deny it
if (!message.guild) { return message.reply('That command may only be used in a server.'); }
// If the command is available only to administrators, run it only if the user is an administrator
if (command.adminOnly) {
if (verifyIsAdmin(message.member)) {
return command.execute(message, args);
} else {
// If the user is not an admin, warn them and bail
return message.author.send("You do not have permission to use that command.");
}
}
// If the command is available to everyone, just run it
if (!command.minimumRole) { return command.execute(message, args); }
// Otherwise, the user must have permission to access this command
if (verifyModeratorRole(message.member)) {
return command.execute(message, args);
}
return message.reply('You are not authorized to use that command.');
}catch (error) {
// Log the error, report a problem
console.error(error);
message.reply("Something broke. Maybe check your command?")
}
});
// Run the voice states through the listeners
client.on('voiceStateUpdate', async(oldState, newState) => {
oldState.member = await cachePartial(oldState.member);
newState.member = await cachePartial(newState.member);
client.voiceStateListeners.forEach((listener) => listener(client, oldState, newState));
});
// Run the reaction updates through the listeners
client.on('messageReactionAdd', async(messageReaction, user) => {
messageReaction = await cachePartial(messageReaction);
messageReaction.message = await cachePartial(messageReaction.message);
client.reactionListeners.forEach((listener) => listener(client, messageReaction, user, true))
});
client.on('messageReactionRemove', async(messageReaction, user) => {
messageReaction = await cachePartial(messageReaction);
messageReaction.message = await cachePartial(messageReaction.message);
client.reactionListeners.forEach((listener) => listener(client, messageReaction, user, false))
});
// Handle the bot being added to a new guild
client.on('guildCreate', async(guild) => handleGuildCreate(client, guild));
// Handle the bot being removed from a guild
client.on('guildDelete', async(guild) => handleGuildDelete(client, guild));
// Use the general error handler to handle unexpected errors
client.on('error', async(error) => generalErrorHandler(error));
client.once('ready', async() => {
await verifyGuildSetups(client);
console.log(`Connected to Discord. Active in ${client.guilds.cache.array().length} guilds.`);
});
client.login(config.token);<file_sep>/messageListeners/fileScanner.js
const {generalErrorHandler} = require('../errorHandlers');
const https = require('https');
const tmp = require('tmp');
const fs = require('fs');
const unZipper = require('unzipper');
const unrar = require('unrar');
const tar = require('tar');
const sevenZip = require('node-7z');
const romExtensions = ['sfc', 'smc', 'rom'];
const archiveExtensions = ['zip', 'rar', 'tar', 'gz', '7z'];
const isRomFile = (filename) => {
const parts = filename.split('.');
for (const part of parts) {
// Rom extension is present in filename
if (romExtensions.indexOf(part) !== -1) { return true; }
}
// Doesn't look like a ROM file
return false;
}
const isArchiveFile = (filename) => {
const parts = filename.split('.');
for (const part of parts) {
// Archive extension is present in filename
if (archiveExtensions.indexOf(part) !== -1) { return true; }
}
// Doesn't look like an archive file
return false;
}
const deleteRomFile = (message) => {
message.channel.send(`${message.author}: Do not post links to ROMs or other copyrighted content.`);
return message.delete();
};
module.exports = (client, message) => {
try{
return message.attachments.each((attachment) => {
// Disallow direct posting of ROM files
if (isRomFile(attachment.name)) {
deleteRomFile(message);
}
if (isArchiveFile(attachment.name)) {
// Download the file so it can be locally analyzed
return https.get(attachment.url, (response) => {
if (response.statusCode !== 200) {
message.channel.send(`${message.author}: Unable to retrieve attachment for analysis. ` +
`Your message has been deleted.`);
message.delete();
return;
}
const tempFile = tmp.fileSync({ postfix: '.aginah', discardDescriptor: true });
const writeStream = fs.createWriteStream(tempFile.name);
response.pipe(writeStream);
writeStream.on('finish', () => {
writeStream.close(() => {
const fileExt = attachment.name.split('.').pop();
let fileDeleted = false;
switch (fileExt) {
case 'zip':
return fs.createReadStream(tempFile.name).pipe(unZipper.Parse())
.on('entry', (file) => {
console.log(file.path+':');
if (isRomFile(file.path) && !fileDeleted) {
console.log('Rom file. Deleting.');
fileDeleted = true;
return deleteRomFile(message);
}
})
case 'rar':
return new unrar(tempFile.name,['n']).list((err, files) => {
if (err) {
console.error(err);
message.channel.send(`${message.author}: Unable to extract file for ` +
"analysis. It has been deleted.");
return message.delete();
}
files.forEach((file) => {
if (fileDeleted) { return; }
if (isRomFile(file.name)) {
fileDeleted = true;
deleteRomFile(message);
}
});
});
case 'tar':
return tar.list({
file: tempFile.name,
onentry: (entry) => {
if (isRomFile(entry.header.path) && !fileDeleted) {
fileDeleted = true;
deleteRomFile(message);
}
},
})
case 'gz':
message.channel.send(`${message.author}: Gzipped files are unsupported. ` +
`Your message has been deleted.`);
return message.delete();
case '7z':
const contents = sevenZip.list(tempFile.name, {
$bin: require('7zip-bin').path7za,
});
return contents.on('data', (data) => {
if (isRomFile(data.file) && !fileDeleted) {
fileDeleted = true;
deleteRomFile(message);
}
});
}
});
// Retain files for one hour, then delete them.
setTimeout(() => {
fs.unlink(tempFile.name, (error) => {if (error) generalErrorHandler(error)});
}, 300 * 1000);
});
}).on('error', (e) => {
message.channel.send(`${message.author}: Unable to retrieve attachment for analysis. ` +
`Your message has been deleted.`);
message.delete();
console.error(e);
});
}
});
} catch (error) {
message.channel.send("Something went wrong while trying to analyze your file. It has been deleted " +
"for safety purposes.");
message.delete();
generalErrorHandler(error);
}
};<file_sep>/commandCategories/roleRequestor.js
const config = require('../config.json');
const {generalErrorHandler} = require('../errorHandlers');
const { parseEmoji, dbQueryOne, dbQueryAll, dbExecute } = require('../lib');
const updateCategoryMessage = async (client, guild, messageId) => {
// Fetch the target message
let sql = `SELECT rc.id, rc.categoryName, rs.roleRequestChannelId FROM role_categories rc
JOIN role_systems rs ON rc.roleSystemId = rs.id
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE gd.guildId=?
AND rc.messageId=?`;
const roleCategory = await dbQueryOne(sql, [guild.id, messageId]);
if (!roleCategory) { throw Error("Unable to update category message. Role category could not be found."); }
const roleInfo = [`> __${roleCategory.categoryName}__`];
sql = `SELECT r.roleId, r.reaction, r.description FROM roles r WHERE r.categoryId=?`;
const roles = await dbQueryAll(sql, [roleCategory.id]);
roles.forEach((role) => {
roleInfo.push(`> ${role.reaction} ${guild.roles.resolve(role.roleId)}` +
`${role.description ? `: ${role.description}` : ''}`);
});
// If there are no roles in this category, mention that there are none
if (roleInfo.length === 1) { roleInfo.push("> There are no roles in this category yet."); }
// Fetch and edit the category message
guild.channels.resolve(roleCategory.roleRequestChannelId).messages.fetch(messageId)
.then((categoryMessage) => categoryMessage.edit(roleInfo)
.then().catch((err) => generalErrorHandler(err)));
};
module.exports = {
category: 'Role Requestor',
commands: [
{
name: 'init-role-system',
description: 'Create a role-request channel for users to interact with AginahBot and request roles.',
longDescription: 'Create a #role-request text channel for users to interact with AginahBot and request ' +
'roles. This channel will be used to post role category messages users can react to to add or ' +
'remove roles.',
aliases: ['irs'],
usage: '`!aginah init-role-system`',
minimumRole: null,
adminOnly: true,
guildOnly: true,
async execute(message) {
let sql = `SELECT gd.id AS guildDataId, rs.id AS roleSystemId
FROM guild_data gd
LEFT JOIN role_systems rs ON gd.id = rs.guildDataId
WHERE gd.guildId=?`;
const row = await dbQueryOne(sql, [message.guild.id]);
if (!row) { throw new Error(); }
if (row.roleSystemId) {
return message.channel.send("The role system has already been set up on your server.");
}
message.guild.channels.create('role-request', {
type: 'text',
topic: 'Request roles so that you may be pinged for various notifications.',
reason: 'Role Request system created.',
permissionOverwrites: [
{
id: message.client.user.id,
allow: [ 'SEND_MESSAGES', 'ADD_REACTIONS' ],
},
{
id: message.guild.roles.everyone.id,
deny: [ 'SEND_MESSAGES', 'ADD_REACTIONS' ]
}
],
}).then(async (channel) => {
// Add role system data to the database
let sql = `INSERT INTO role_systems (guildDataId, roleRequestChannelId) VALUES(?, ?)`
await dbExecute(sql, [row.guildDataId, channel.id]);
channel.send(`The following roles are available on this server. If you would like to be ` +
`assigned a role, please react to the appropriate message with the indicated ` +
`emoji. All roles are pingable by everyone on the server. Remember, with great ` +
`power comes great responsibility.`);
}).catch((error) => generalErrorHandler(error));
}
},
{
name: 'destroy-role-system',
description: 'Delete the role-request channel and all categories and permissions created by this bot.',
longDescription: null,
aliases: ['drs'],
usage: '`!aginah destroy-role-system`',
minimumRole: null,
adminOnly: true,
guildOnly: true,
async execute(message) {
let sql = `SELECT rs.id, rs.roleRequestChannelId FROM role_systems rs
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE gd.guildId=?`;
const roleSystem = await dbQueryOne(sql, [message.guild.id]);
if (!roleSystem) {
// If the role system does not exist, there is no need to attempt to delete it
return message.channel.send("The role system is not currently installed on this server.");
}
// Loop over the role categories and delete them
sql = `SELECT id FROM role_categories WHERE roleSystemId=?`;
const roleCategories = await dbQueryAll(sql, [roleSystem.id]);
for (let roleCategory of roleCategories) {
// Loop over the roles in each category and delete them from the server
const roles = await dbQueryAll(`SELECT roleId FROM roles WHERE categoryId=?`, [roleCategory.id]);
for (let role of roles) {
await message.guild.roles.resolve(role.roleId).delete();
}
await dbExecute(`DELETE FROM roles WHERE categoryId=?`, [roleCategory.id]);
}
// Database cleanup
await dbExecute(`DELETE FROM role_categories WHERE roleSystemId=?`, [roleSystem.id]);
message.guild.channels.resolve(roleSystem.roleRequestChannelId).delete();
await dbExecute(`DELETE FROM role_systems WHERE id=?`, [roleSystem.id]);
}
},
{
name: 'create-role-category',
description: 'Create a category for roles to be added to.',
longDescription: `Create a category for roles to be added to. Each category will have its own message ` +
`in the #role-request channel. Category names must be a single alphanumeric word.`,
aliases: [],
usage: '`!aginah create-role-category CategoryName`',
minimumRole: config.moderatorRole,
adminOnly: false,
guildOnly: true,
async execute(message, args) {
if (args.length === 0) {
return message.channel.send("You must specify a name for the category!");
}
let sql = `SELECT 1 FROM role_categories rc
JOIN role_systems rs ON rc.roleSystemId = rs.id
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE gd.guildId=?
AND rc.categoryName=?`;
const row = await dbQueryOne(sql, [message.guild.id, args[0]]);
if (row) { return message.channel.send("That category already exists!"); }
sql = `SELECT rs.id, rs.roleRequestChannelId
FROM role_systems rs
JOIN guild_data gd ON rs.guildDataId=gd.id
WHERE gd.guildId=?`;
const roleSystem = await dbQueryOne(sql, [message.guild.id]);
if (!roleSystem) {
return message.channel.send("The role system has not been setup on this server.");
}
// Add the category message to the #role-request channel
message.guild.channels.resolve(roleSystem.roleRequestChannelId).send("Creating new category...")
.then(async (categoryMessage) => {
// Update database with new category message data
let sql = `INSERT INTO role_categories (roleSystemId, categoryName, messageId) VALUES (?, ?, ?)`;
await dbExecute(sql, [roleSystem.id, args[0], categoryMessage.id]);
await updateCategoryMessage(message.client, message.guild, categoryMessage.id);
});
}
},
{
name: 'delete-role-category',
description: 'Delete a role category.',
longDescription: 'Delete a role category. All roles within this category will also be deleted.',
aliases: [],
usage: '`!aginah delete-role-category CategoryName`',
minimumRole: config.moderatorRole,
adminOnly: false,
guildOnly: true,
async execute(message, args) {
if (args.length === 0) {
return message.channel.send("You must specify the category to delete!");
}
// Get the role category data
let sql = `SELECT rc.id, rc.messageId, rs.roleRequestChannelId FROM role_categories rc
JOIN role_systems rs ON rc.roleSystemId = rs.id
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE gd.guildId=?
AND rc.categoryName=?`;
const roleCategory = await dbQueryOne(sql, [message.guild.id, args[0]]);
if (!roleCategory) { return message.channel.send("That category does not exist!"); }
await dbExecute(`DELETE FROM roles WHERE categoryId=?`, [roleCategory.id]);
await dbExecute(`DELETE FROM role_categories WHERE id=?`, [roleCategory.id]);
message.guild.channels.resolve(roleCategory.roleRequestChannelId).messages.fetch(roleCategory.messageId)
.then((categoryMessage) => categoryMessage.delete()).catch((err) => generalErrorHandler(err));
}
},
{
name: 'create-role',
description: 'Create a pingable role.',
longDescription: null,
aliases: [],
usage: '`!aginah cmd create-role CategoryName RoleName Reaction [Description]`',
minimumRole: config.moderatorRole,
adminOnly: false,
guildOnly: true,
async execute(message, args) {
// Check for existing role
let sql = `SELECT 1 FROM roles r
JOIN role_categories rc ON r.categoryId = rc.id
JOIN role_systems rs ON rc.roleSystemId = rs.id
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE gd.guildId=?
AND rc.categoryName=?
AND r.roleName=?`;
const row = await dbQueryOne(sql, [message.guild.id, args[0], args[1]]);
// If the role already exists, do nothing
if (row) { return message.channel.send("That role already exists!"); }
// Verify the requested emoji is available on this server
const emoji = parseEmoji(message.guild, args[2]);
if (!emoji) { return message.channel.send("That emoji is not available on this server!"); }
sql = `SELECT rc.id, rc.messageId, rs.roleRequestChannelId FROM role_categories rc
JOIN role_systems rs ON rc.roleSystemId = rs.id
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE gd.guildId=?
AND rc.categoryName=?`;
const roleCategory = await dbQueryOne(sql, [message.guild.id, args[0]]);
// If there is no such category, warn the user and do nothing
if (!roleCategory) { return message.channel.send("That category doesn't exist!"); }
// Create the role on the server
message.guild.roles.create({
data: {
name: args[1],
mentionable: true,
},
reason: 'Added as part of role-request system.',
}).then(async (role) => {
// Add the role to the database
await dbExecute(
`INSERT INTO roles (categoryId, roleId, roleName, reaction, description) VALUES (?, ?, ?, ?, ?)`,
[roleCategory.id, role.id, args[1], emoji.toString(), (args[3] ? args.slice(3).join(' ') : null)]
);
await updateCategoryMessage(message.client, message.guild, roleCategory.messageId);
// Add the reaction to the category message
message.guild.channels.resolve(roleCategory.roleRequestChannelId).messages.fetch(roleCategory.messageId)
.then((categoryMessage) => categoryMessage.react(emoji).catch((err) => generalErrorHandler(err)));
}).catch((error) => {
throw new Error(`Unable to create guild role. ${error}`);
});
}
},
{
name: 'modify-role-reaction',
description: 'Alter the reaction associated with a role created by this bot.',
longDescription: null,
aliases: [],
usage: '`!aginah modify-role-reaction CategoryName RoleName Reaction`',
minimumRole: config.moderatorRole,
adminOnly: false,
guildOnly: true,
async execute(message, args) {
// Check for existing role
let sql = `SELECT r.id, rc.messageId, rs.roleRequestChannelId, r.reaction FROM roles r
JOIN role_categories rc ON r.categoryId = rc.id
JOIN role_systems rs ON rc.roleSystemId = rs.id
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE gd.guildId=?
AND rc.categoryName=?
AND r.roleName=?`;
const role = await dbQueryOne(sql, [message.guild.id, args[0], args[1]]);
// If the role already exists, do nothing
if (!role) { return message.channel.send("That role does not exist!"); }
// Verify the requested emoji is available on this server
const emoji = parseEmoji(message.guild, args[2]);
if (!emoji) { return message.channel.send("That emoji is not available on this server!"); }
// Remove reactions from the role category message
message.guild.channels.resolve(role.roleRequestChannelId).messages.fetch(role.messageId)
.then((categoryMessage) => {
categoryMessage.reactions.cache.each((reaction) => {
if (reaction.emoji.toString() === role.reaction) { reaction.remove(); }
});
// Add new reaction to message
categoryMessage.react(emoji);
}).catch((err) => generalErrorHandler(err));
await dbExecute(`UPDATE roles SET reaction=? WHERE id=?`, [emoji.toString(), role.id]);
await updateCategoryMessage(message.client, message.guild, role.messageId);
}
},
{
name: 'modify-role-description',
description: 'Alter the description associated with a role created by this bot.',
longDescription: null,
aliases: [],
usage: '`!aginah modify-role-description CategoryName RoleName [Description]`',
minimumRole: config.moderatorRole,
adminOnly: false,
guildOnly: true,
async execute(message, args) {
// Check for existing role
let sql = `SELECT r.id, rc.messageId, rs.roleRequestChannelId FROM roles r
JOIN role_categories rc ON r.categoryId = rc.id
JOIN role_systems rs ON rc.roleSystemId = rs.id
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE gd.guildId=?
AND rc.categoryName=?
AND r.roleName=?`;
const role = await dbQueryOne(sql, [message.guild.id, args[0], args[1]]);
if (!role) { return message.channel.send("That role does not exist!"); }
sql = `UPDATE roles SET description=? WHERE id=?`;
await dbExecute(sql, [(args[2] ? args.slice(2).join(' ') : null), role.id]);
await updateCategoryMessage(message.client, message.guild, role.messageId);
}
},
{
name: 'delete-role',
description: 'Delete a role created by this bot.',
longDescription: null,
aliases: [],
usage: '`!aginah delete-role CategoryName RoleName`',
minimumRole: config.moderatorRole,
adminOnly: false,
guildOnly: true,
async execute(message, args) {
let sql = `SELECT r.id, rc.id AS categoryId, rc.messageId, r.reaction, r.roleId, rs.roleRequestChannelId
FROM roles r
JOIN role_categories rc ON r.categoryId = rc.id
JOIN role_systems rs ON rc.roleSystemId = rs.id
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE gd.guildId=?
AND rc.categoryName=?
AND r.roleName=?`;
const role = await dbQueryOne(sql, [message.guild.id, args[0], args[1]]);
if (!role) { return message.channel.send("That role does not exist!"); }
// Remove reactions from the role category message
message.guild.channels.resolve(role.roleRequestChannelId).messages.fetch(role.messageId)
.then((categoryMessage) => categoryMessage.reactions.cache.array().forEach((r) => {
if (r.emoji.toString() === role.reaction) { r.remove(); }
}))
.catch((err) => generalErrorHandler(err));
// Remove the role from the guild
message.guild.roles.resolve(role.roleId).delete();
// Delete rows from the roles table and update role category message
await dbExecute(`DELETE FROM roles WHERE id=? AND categoryId=?`, [role.id, role.categoryId]);
await updateCategoryMessage(message.client, message.guild, role.messageId);
}
},
],
};
<file_sep>/commandCategories/helpfulMessages.js
const { supportedGames } = require('../assets/supportedGames.json');
module.exports = {
category: 'Helpful Messages',
commands: [
{
name: 'setup',
description: 'Get instructions on how to start playing games!',
longDescription: null,
aliases: ['setup-guide'],
usage: '`!aginah setup game`',
guildOnly: false,
minimumRole: null,
adminOnly: false,
execute(message, args) {
if (args.length === 0) {
return message.channel.send("You must specify which game to get the setup guide for. " +
"Use `!aginah games` to get a list of available games.");
}
if (!supportedGames.hasOwnProperty(args[0].toLowerCase())) {
return message.channel.send("There is no setup guide available for that game.");
}
return message.channel.send(`The setup guide may be found here:\n` +
`${supportedGames[args[0].toLowerCase()].setupGuide}`);
}
},
{
name: 'website',
description: 'Get the link to a game\'s website.',
longDescription: null,
aliases: ['site', 'webpage'],
usage: '`!aginah website game`',
guildOnly: false,
minimumRole: null,
adminOnly: false,
execute(message, args) {
if (args.length === 0) {
return message.channel.send("You must specify which game to get the website for. " +
"Use `!aginah games` to get a list of available games.");
}
if (!supportedGames.hasOwnProperty(args[0].toLowerCase())) {
return message.channel.send("There is no website available for that game.");
}
return message.channel.send(`The website may be found here:\n` +
`${supportedGames[args[0].toLowerCase()].website}`);
}
},
{
name: 'code',
description: 'Get links to the code repositories associated with a game.',
longDescription: null,
aliases: ['github', 'git'],
usage: '`!aginah code game`',
guildOnly: false,
minimumRole: null,
adminOnly: false,
execute(message, args) {
if (args.length === 0) {
return message.channel.send("The AginahBot code may be found here:\n" +
"https://github.com/LegendaryLinux/AginahBot");
}
if (!supportedGames.hasOwnProperty(args[0].toLowerCase())) {
return message.channel.send("There are no code repositories available for that game.");
}
const game = supportedGames[args[0].toLowerCase()];
return message.channel.send([
`The code repositories associated with the ${game.friendlyName} project are:`,
...game.repositories
]);
}
},
],
};<file_sep>/README.md
# AginahBot
A Discord bot designed to help organize and moderate Multiplayer-Capable Randomizer games.
Find it in use at the [MultiWorld Discord](https://discord.gg/B5pjMYy).
[Click here to learn how to add AginahBot to your Discord server!](https://github.com/LegendaryLinux/AginahBot/wiki/Using-AginahBot-on-Your-Discord-Server)
## Current Features
- Support dynamic voice and text channels, and automatic assignment of permissions on those channels
- Ready check system for users in dynamic game channels
- Privacy controls for users in dynamic game channels
- Automatically delete ROM files, and compressed files containing them
- Organization features to help schedule games
- Alert players in game lobbies that their seeds are ready
- Custom role system to allow users to assign themselves pingable roles to be alerted of games
- Generate single-player or multiplayer games using the `generate` command
## Supported Games
- The Legend of Zelda: A Link to the Past
# Self-Hosting
## Prerequisites
- `node` and `npm` should be installed to run the bot and install dependencies
- `unrar` should be installed on your system to process `.rar` files.
- A MySQL 8 server should be installed, and a database available.
## Configuration
A `config.json` file is required to be present in the base directory of the repository. This file should contain
your Discord bot's secret key, database information, a command prefix, and a name for the Moderator role which
will be created on servers the bot joins if it does not exist already.
Example config:
```json
{
"token": "discord-bot-token",
"dbHost": "hostname-of-mysql-server",
"dbUser": "database-username",
"dbPass": "<PASSWORD>",
"dbName": "database-name",
"commandPrefix": "!aginah ",
"moderatorRole": "Moderator"
}
```
If you intend to create your own bot on Discord using the code in this repository, your bot will need
permissions granted by the permissions integer `285273168`.
The following permissions will be granted
to AginahBot:
- Manage Roles
- Manage Channels
- Read Text Channels & See Voice Channels
- Send Messages
- Manage Messages
- Embed Links
- Attach Files
- Add Reactions
- Move Members (Voice Channels)
## Setup
```shell script
# Clone the repo
git clone https://github.com/LegendaryLinux/AginahBot
# Enter its directory
cd AginahBot
# Install required packages
npm install
# Set up your config.json file
vim config.json
# Initialize the database
node dbSetup.js
# Run the bot
node bot.js
```
Note that `dbSetup.js` requires a database user with the `CREATE TABLE` permission, but normal operation requires
only `SELECT`, `INSERT`, `UPDATE`, and `DELETE` on your target database. It is recommended to use an administrative
user to configure the database, and a non-admin user with restricted permissions during normal operation.<file_sep>/commandCategories/scheduling.js
const {generalErrorHandler} = require('../errorHandlers');
const moment = require('moment-timezone');
const { dbQueryOne, dbQueryAll, dbExecute } = require('../lib');
// Return the offset in hours of a given timezone
const getZoneOffset = (zone) => 0 - moment.tz('1970-01-01 00:00', zone).toDate().getTime() / 1000 / 60 / 60;
const months = { 0: 'January', 1: 'February', 2: 'March', 3: 'April', 4: 'May', 5: 'June', 6: 'July', 7: 'August',
8: 'September', 9: 'October', 10: 'November', 11: 'December' };
const sendScheduleMessage = async (message, targetDate) => message.channel.send([
`${message.author} wants to schedule a game for ` +
`${months[targetDate.getUTCMonth()]} ${targetDate.getUTCDate()}, ${targetDate.getUTCFullYear()} at ` +
`${targetDate.getUTCHours()}:${targetDate.getUTCMinutes().toString().padStart(2,'0')} UTC`,
`https://gametimes.multiworld.link/?timestamp=${targetDate.getTime()}`,
'React with ⚔ if you intend to join this game.',
'React with 🐔 if you don\'t know yet.'
]).then(async (scheduleMessage) => {
// Save scheduled game to database
const guildData = await dbQueryOne(`SELECT id FROM guild_data WHERE guildId=?`, [message.guild.id]);
if (!guildData) {
throw new Error(`Unable to find guild ${message.guild.name} (${message.guild.id}) in guild_data table.`);
}
let sql = `INSERT INTO scheduled_events
(guildDataId, timestamp, channelId, messageId, schedulingUserId, schedulingUserTag)
VALUES (?, ?, ?, ?, ?, ?)`;
await dbExecute(sql, [guildData.id, targetDate.getTime(), scheduleMessage.channel.id, scheduleMessage.id,
message.member.user.id, message.member.user.tag]);
// Put appropriate reactions onto the message
scheduleMessage.react('⚔');
scheduleMessage.react('🐔');
}).catch((error) => generalErrorHandler(error));
module.exports = {
category: 'Event Scheduling',
commands: [
{
name: 'schedule',
description: 'View upcoming events or schedule a new one',
longDescription: "View upcoming events or Schedule a new one. Allowed times look like:\n\n" +
"`X:00`: Schedule a game for the next occurrence of the provided minutes value\n" +
"`HH:MM TZ`: Schedule a game for the next occurrence of the provided time.\n" +
"`MM/DD/YYYY HH:MM TZ`: Schedule a game for the specific provided date and time.\n" +
"`YYYY-MM-DD HH:MM TZ` Schedule a game for a specific provided date and time.\n\n" +
"Strict ISO-8601 formatted datetime values are also allowed.\n" +
"Users subject to daylight savings time, be aware you may have two different timezones. EST / EDT, " +
"for example.\n",
aliases: [],
usage: '`!aginah schedule [role date/time]`',
guildOnly: true,
minimumRole: null,
adminOnly: false,
async execute(message, args) {
if (args.length === 0) {
let sql = `SELECT se.timestamp, se.schedulingUserTag, se.channelId, se.messageId,
(SELECT COUNT(*) FROM event_attendees WHERE eventId=se.id) AS rsvpCount
FROM scheduled_events se
JOIN guild_data gd ON se.guildDataId = gd.id
WHERE gd.guildId=?
AND se.timestamp > ?`;
const games = await dbQueryAll(sql, [message.guild.id, new Date().getTime()]);
for (let game of games) {
const channel = message.guild.channels.resolve(game.channelId);
if (!channel) { continue; }
channel.messages.fetch(game.messageId).then(
(scheduleMessage) => {
const gameTime = new Date(parseInt(game.timestamp, 10));
message.channel.send(
`> **${game.schedulingUserTag}** scheduled a game for **` +
`${gameTime.getUTCMonth()+1}/${gameTime.getUTCDate()}/` +
`${gameTime.getUTCFullYear()} ${gameTime.getUTCHours()}:` +
`${gameTime.getUTCMinutes().toString().padStart(2, '0')} UTC**.\n` +
`> In your timezone: ` +
`https://gametimes.multiworld.link/` +
`?timestamp=${parseInt(game.timestamp, 10)}\n` +
`> RSVP Link: ${scheduleMessage.url}\n` +
`> Current RSVPs: ${game.rsvpCount}`);
}).catch((err) => generalErrorHandler(err));
}
if (games.length === 0) { return message.channel.send("There are currently no games scheduled."); }
return;
}
if (args.length < 2) {
return message.channel.send("Looks like you're missing some arguments. Use " +
"`!aginah help schedule` for more info.");
}
// Remove the role argument, since we don't do anything with it
await args.shift();
const timeString = args.join(' ').toUpperCase().trim();
const currentDate = new Date();
// Format: Strict ISO-8601
const iso8601Pattern = new RegExp(/^\d{4}-\d{2}-\d{2}T\d{2}:\d{2}(Z|([+-]\d{2}:\d{2}))$/);
// Format: MM/DD/YYYY HH:II TZ
const mdyPattern = new RegExp(/^(\d{1,2})\/(\d{1,2})\/(\d{4}) (\d{1,2}):(\d{2}) ([A-z]*)$/);
// Format: YYYY-MM-DD HH:MM TZ
const isoSimplePattern = new RegExp(/^(\d{4})-(\d{1,2})-(\d{1,2}) (\d{1,2}):(\d{2}) ([A-z]*)$/);
// Format: HH:MM TZ
const specificHourPattern = new RegExp(/^(\d{1,2}):(\d{2}) ([A-z]*)$/);
// Format XX:30
const nextHourPattern = new RegExp(/^X{1,2}:(\d{2})$/);
if (timeString.search(iso8601Pattern) > -1) {
const targetDate = new Date(timeString);
if (isNaN(targetDate.getTime())) {
return message.channel.send("The date you provided is invalid.");
}
if (targetDate.getTime() < currentDate.getTime()) {
return message.channel.send("You can't schedule a game in the past!");
}
return sendScheduleMessage(message, targetDate);
} else if (timeString.search(mdyPattern) > -1) {
const patternParts = timeString.match(mdyPattern);
if (!moment.tz.zone(patternParts[6])) {
return message.channel.send("I don't recognize that timezone! Does your timezone " +
"change for daylight savings time?");
}
const targetDate = new Date(timeString);
if (isNaN(targetDate.getTime())) {
return message.channel.send("The date you provided is invalid.");
}
if (targetDate.getTime() < currentDate.getTime()) {
return message.channel.send("You can't schedule a game in the past!");
}
return sendScheduleMessage(message, targetDate);
} else if (timeString.search(isoSimplePattern) > -1) {
const patternParts = timeString.match(isoSimplePattern);
if (!moment.tz.zone(patternParts[6])) {
return message.channel.send("I don't recognize that timezone! Does your timezone " +
"change for daylight savings time?");
}
const zoneOffset = getZoneOffset(patternParts[6]);
if (isNaN(zoneOffset)) {
return message.channel.send("I couldn't schedule your game because the timezone provided " +
"could not be used to create a valid date object.");
}
const sign = zoneOffset < 1 ? '-' : '+';
const targetDate = new Date(`${patternParts[1]}-${patternParts[2]}-${patternParts[3]}T` +
`${patternParts[4]}:${patternParts[5]}${sign}` +
`${Math.abs(zoneOffset).toString().padStart(2, '0')}:00`);
if (targetDate.getTime() < currentDate.getTime()) {
return message.channel.send("You can't schedule a game in the past!");
}
return sendScheduleMessage(message, targetDate);
} else if (timeString.search(specificHourPattern) > -1) {
const patternParts = timeString.match(specificHourPattern);
if (parseInt(patternParts[1], 10) > 24) {
return message.channel.send("There are only 24 hours in a day!");
}
if (parseInt(patternParts[2], 10) > 59) {
return message.channel.send("There are only 60 minutes in an hour!");
}
if (!moment.tz.zone(patternParts[3])) {
return message.channel.send("I don't recognize that timezone! Does your timezone " +
"change for daylight savings time?");
}
const zoneOffset = getZoneOffset(patternParts[3]);
if (isNaN(zoneOffset)) {
return message.channel.send("I couldn't schedule your game because the timezone provided " +
"could not be used to create a valid date object.");
}
const targetDate = new Date(currentDate.getTime());
targetDate.setUTCHours(parseInt(patternParts[1], 10));
targetDate.setUTCMinutes(parseInt(patternParts[2], 10));
targetDate.setUTCSeconds(0);
targetDate.setUTCMilliseconds(0);
targetDate.setTime(targetDate.getTime() - (zoneOffset * 60 * 60 * 1000));
while (targetDate.getTime() < currentDate.getTime()) {
targetDate.setTime(targetDate.getTime() + (24 * 60 * 60 * 1000));
}
return sendScheduleMessage(message, targetDate);
} else if (timeString.search(nextHourPattern) > -1) {
const patternParts = timeString.match(nextHourPattern);
if (patternParts[1] > 59) {
return message.channel.send("There are only sixty minutes in an hour!");
}
const targetDate = new Date(`${currentDate.getUTCMonth()+1}/${currentDate.getUTCDate()}`+
`/${currentDate.getUTCFullYear()} ${currentDate.getUTCHours()}:${patternParts[1]} UTC`);
if (targetDate.getTime() < currentDate.getTime()) {
targetDate.setUTCHours(targetDate.getUTCHours() + 1);
}
return sendScheduleMessage(message, targetDate);
} else {
return message.channel.send("Sorry, I don't understand that time. Use " +
"`!aginah help schedule` for more info.");
}
}
},
],
};<file_sep>/commandCategories/gameGenerator.js
const axios = require('axios');
const request = require('request');
const FormData = require('form-data');
const jsYaml = require('js-yaml');
const tmp = require('tmp');
const fs = require('fs');
const { supportedGames } = require('../assets/supportedGames.json');
const { presets } = require('../assets/presets.json');
module.exports = {
category: 'Game Generator',
commands: [
{
name: 'generate',
description: 'Generate a game based on a preset or uploaded file.',
longDescription: null,
aliases: ['gen'],
usage: `!aginah generate game preset|configFile [race] [playerCount]`,
guildOnly: false,
minimumRole: null,
adminOnly: false,
execute(message, args) {
if (args.length === 0) {
return message.channel.send("You must specify a game to generate.");
}
// A game must be specified
if (!supportedGames.hasOwnProperty(args[0].toLowerCase())) {
return message.channel.send("Sorry that game isn't supported yet. `!aginah games` for more info.");
}
// Handle requests to generate a game from a file
if (message.attachments.array().length > 0){
// Argument validation. Arguments 1 and 2 can be provided in any order, or not at all.
// Here, they are parsed if present and their meanings interpreted. The word "race" indicates
// a race request while a number indicates a player count.
let playerCount = '1';
let race = '0';
[1, 2].forEach((index) => {
if (!args[index]) { return; }
if (args[index].toLowerCase() === 'race') { race = '1'; }
if (args[index].search(/^\d+$/) > -1) { playerCount = args[index].toString(); }
});
const postfix = '.'+message.attachments.array()[0].name.split('.').reverse()[0];
const tempFile = tmp.fileSync({ prefix: "upload-", postfix });
return request.get(message.attachments.array()[0].url)
.pipe(fs.createWriteStream(tempFile.name))
.on('close', () => {
// Send request to api
const formData = new FormData();
formData.append('file', fs.createReadStream(tempFile.name), tempFile.name);
formData.append('playerCount', playerCount);
formData.append('race', race);
const axiosOpts = { headers: formData.getHeaders() };
axios.post(supportedGames[args[0].toLowerCase()].apiEndpoint, formData, axiosOpts)
.then((bResponse) => { // Berserker Response
message.channel.send(`Seed generation underway. When it's ready, you will be ` +
`able to download your patch file from:\n${bResponse.data.url}`);
tempFile.removeCallback();
}).catch((error) => {
message.channel.send("I couldn't generate that game, sorry.");
if(error.isAxiosError && error.response.data){
console.error(`Unable to generate Z3 game on ${API_ENDPOINT}. The following ` +
`data was returned from the endpoint:`);
return console.error(error.response.data);
}
return console.error(error);
});
});
}
// Handle requests to generate a game from a preset. Required arguments must be present
if (args.length < 2) {
return message.channel.send("You must specify a game and preset to generate.");
}
// Game must exist in presets object
if (args[0] && !presets.hasOwnProperty(args[0].toLowerCase())) {
return message.channel.send("Presets are not currently supported for that game.");
}
// Presets must exist in presets.game object
if (!presets[args[0].toLowerCase()].hasOwnProperty(args[1].toLowerCase())) {
return message.channel.send("That preset doesn't exist for the requested game.");
}
// Argument validation. Similar to the above validation, but the argument positions are increased
// by one. Arguments 2 and 3 can be provided in any order, or not at all.
// Here, they are parsed if present and their meanings interpreted. The word "race" indicates
// a race request while a number indicates a player count.
let playerCount = '1';
let race = '0';
[2, 3].forEach((index) => {
if (!args[index]) { return; }
if (args[index].toLowerCase() === 'race') { race = '1'; }
if (args[index].search(/^\d+$/) > -1) { playerCount = args[index].toString(); }
});
return axios.post(supportedGames[args[0].toLowerCase()].apiEndpoint, {
weights: { [args[1].toLowerCase()]: presets[args[0].toLowerCase()][args[1].toLowerCase()] },
presetData: { [args[1].toLowerCase()]: presets[args[0].toLowerCase()][args[1].toLowerCase()] },
playerCount,
race,
}).then((bResponse) => {
message.channel.send(`Seed generation underway. When it's ready, you will be able to ` +
`download your patch file from:\n${bResponse.data.url}`);
}).catch((error) => {
message.channel.send("I couldn't generate that game, sorry.");
if(error.isAxiosError && error.response.data){
console.error(`Unable to generate game. The following data was returned from the endpoint:`);
return console.error(error.response.data);
}
return console.error(error);
});
}
},
{
name: 'presets',
description: 'Get a list of presets or download a preset file.',
longDescription: null,
aliases: [],
usage: '`!aginah presets game [preset]`',
guildOnly: false,
minimumRole: null,
adminOnly: false,
execute(message, args) {
if (args.length === 0) {
return message.channel.send("You must specify a game to list presets for. Use " +
"`!aginah help games` to get a list of supported games.");
}
if (!presets.hasOwnProperty(args[0].toLowerCase())) {
return message.channel.send("Presets have not been configured for that game.");
}
if (args.length === 1) {
const response = ['The following presets are available:'];
Object.keys(presets[args[0].toLowerCase()]).forEach((preset) => response.push(` - ${preset}`));
return message.channel.send(response);
}
if (!presets[args[0].toLowerCase()].hasOwnProperty(args[1].toLowerCase())) {
return message.channel.send("I don't know that preset. Use `!aginah game presets` to get a list " +
"of available presets.");
}
const preferYaml = supportedGames[args[0].toLowerCase()].preferYaml;
let presetData = null;
if (preferYaml){
const yamlOpts = { noCompatMode: true };
presetData = jsYaml.safeDump(presets[args[0].toLowerCase()][args[1].toLowerCase()], yamlOpts)
.replace(/'(\d+)':/g, (x, y) => `${y}:`);
} else {
presetData = JSON.stringify(presets[args[0].toLowerCase()][args[1].toLowerCase()]);
}
return tmp.file((err, tmpFilePath, fd, cleanupCallback) => {
fs.writeFile(tmpFilePath, presetData, () => {
return message.channel.send({
files: [
{
name: args[1].toLowerCase()+(preferYaml ? '.yaml' : '.json'),
attachment: tmpFilePath,
}
],
}).then(() => cleanupCallback);
});
});
}
},
{
name: 'games',
description: 'Get a list of games AginahBot can generate seeds for.',
longDescription: null,
aliases: [],
usage: '`!aginah games`',
guildOnly: false,
minimumRole: null,
adminOnly: false,
execute(message) {
const data = ['The currently supported games are:'];
Object.keys(supportedGames).forEach((game) => {
data.push(` - ${supportedGames[game].friendlyName} (**${game}**)`);
});
return message.channel.send(data);
}
}
],
};<file_sep>/lib.js
const Discord = require('discord.js');
const mysql = require('mysql2');
const config = require('./config.json');
const { generalErrorHandler } = require('./errorHandlers');
module.exports = {
// Function which returns a promise which will resolve to true or false
verifyModeratorRole: (guildMember) => {
if (module.exports.verifyIsAdmin(guildMember)) { return true; }
return module.exports.getModeratorRole(guildMember.guild).position <= guildMember.roles.highest.position;
},
verifyIsAdmin: (guildMember) => guildMember.hasPermission(Discord.Permissions.FLAGS.ADMINISTRATOR),
getModeratorRole: (guild) => {
// Find this guild's moderator role
for (const role of guild.roles.cache.array()) {
if (role.name === config.moderatorRole) {
return role;
}
}
return null;
},
handleGuildCreate: async (client, guild) => {
// Find this guild's moderator role id
let moderatorRole = module.exports.getModeratorRole(guild);
if (!moderatorRole) {
return guild.roles.create({
data: {
name: config.moderatorRole,
reason: `AginahBot requires a ${config.moderatorRole} role.`
},
}).then(async (moderatorRole) => {
let sql = `INSERT INTO guild_data (guildId, moderatorRoleId) VALUES (?, ?)`;
await module.exports.dbExecute(sql, [guild.id, moderatorRole.id]);
}).catch((err) => generalErrorHandler(err));
}
let sql = `INSERT INTO guild_data (guildId, moderatorRoleId) VALUES (?, ?)`;
await module.exports.dbExecute(sql, [guild.id, moderatorRole.id]);
},
handleGuildDelete: async (client, guild) => {
const guildData = await module.exports.dbQueryOne(`SELECT id FROM guild_data WHERE guildId=?`, [guild.id])
if (!guildData) {
throw new Error(`No guild data could be found when trying to delete data for guild:` +
`${guild.name} (${guild.id}).`);
}
// Delete dynamic game system data
const roomSystems = await module.exports.dbQueryAll(`SELECT id FROM room_systems WHERE guildDataId=?`,
[guildData.id]);
roomSystems.forEach((roomSystem) => {
module.exports.dbExecute(`DELETE FROM room_system_games WHERE roomSystemId=?`, [roomSystem.id]);
module.exports.dbExecute(`DELETE FROM room_systems WHERE id=?`, [roomSystem.id]);
});
// Delete role requestor system data
const roleSystem = await module.exports.dbQueryOne(`SELECT id FROM role_systems WHERE guildDataId=?`,
[guildData.id]);
if (roleSystem) {
const categories = await module.exports.dbQueryAll(`SELECT id FROM role_categories WHERE roleSystemId=?`,
[roleSystem.id]);
categories.forEach((category) => {
module.exports.dbExecute(`DELETE FROM roles WHERE categoryId=?`, [category.id]);
});
await module.exports.dbExecute(`DELETE FROM role_categories WHERE roleSystemId=?`, [roleSystem.id]);
await module.exports.dbExecute(`DELETE FROM role_systems WHERE id=?`, [roleSystem.id]);
}
// Delete guild data
await module.exports.dbExecute(`DELETE FROM guild_data WHERE id=?`, [guildData.id]);
},
verifyGuildSetups: async (client) => {
client.guilds.cache.each(async (guild) => {
const row = await module.exports.dbQueryOne(`SELECT 1 FROM guild_data WHERE guildId=?`, [guild.id]);
if (!row) { await module.exports.handleGuildCreate(client, guild); }
});
},
/**
* Get an emoji object usable with Discord. Null if the Emoji is not usable in the provided guild.
* @param guild
* @param emoji
* @returns String || Object || null
*/
parseEmoji: (guild, emoji) => {
const emojiIdRegex = new RegExp(/^<:.*:(\d+)>$/);
const match = emoji.match(emojiIdRegex);
if (match && match.length > 1) {
const emojiObj = guild.emojis.resolve(match[1]);
return emojiObj ? emojiObj : null;
}
const nodeEmoji = require('node-emoji');
return nodeEmoji.hasEmoji(emoji) ? emoji : null;
},
cachePartial: (partial) => new Promise((resolve, reject) => {
if (!partial.hasOwnProperty('partial') || !partial.partial) { resolve(partial); }
partial.fetch()
.then((full) => resolve(full))
.catch((error) => reject(error));
}),
dbConnect: () => mysql.createConnection({
host: config.dbHost,
user: config.dbUser,
password: <PASSWORD>,
database: config.dbName,
supportBigNumbers: true,
bigNumberStrings: true,
}),
dbQueryOne: (sql, args) => new Promise((resolve, reject) => {
const conn = module.exports.dbConnect();
conn.query(sql, args, (err, result) => {
if (err) { reject(err); }
else if (result.length > 1) { reject('More than one row returned'); }
else { resolve(result.length === 1 ? result[0] : null); }
return conn.end();
});
}),
dbQueryAll: (sql, args) => new Promise((resolve, reject) => {
const conn = module.exports.dbConnect();
conn.query(sql, args, (err, result) => {
if (err) { reject(err); }
else { resolve(result); }
return conn.end();
});
}),
dbExecute: (sql, args) => new Promise((resolve, reject) => {
const conn = module.exports.dbConnect();
conn.execute(sql, args, (err) => {
if (err) { reject(err); }
else { resolve(); }
return conn.end();
});
}),
};
<file_sep>/messageListeners/roomSystemCommands.js
const { getModeratorRole, dbQueryOne, dbQueryAll, dbExecute } = require('../lib');
module.exports = async (client, message) => {
if (!message.guild) { return; }
const commands = ['.ready', '.unready', '.readycheck', '.close', '.lock', '.unlock'];
if (commands.indexOf(message.content) === -1) { return; }
let sql = `SELECT rsrc.id AS checkId, rsg.id AS gameId, rsg.voiceChannelId, rs.planningChannelId
FROM room_system_ready_checks rsrc
JOIN room_system_games rsg ON rsrc.gameId = rsg.id
JOIN room_systems rs ON rsg.roomSystemId = rs.id
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE rsrc.playerId=?
AND rsg.textChannelId=?
AND gd.guildId=?`
const roomSystem = await dbQueryOne(sql, [message.author.id, message.channel.id, message.guild.id]);
if (!roomSystem) { return; }
switch(message.content) {
// Player has indicated they are ready to begin
case '.ready':
return dbExecute(`UPDATE room_system_ready_checks SET readyState=1 WHERE id=?`, [roomSystem.checkId]);
// Player has indicated they are no longer ready to begin
case '.unready':
return dbExecute(`UPDATE room_system_ready_checks SET readyState=0 WHERE id=?`, [roomSystem.checkId]);
// Print a list of players who are and are not ready
case '.readycheck':
const ready = [];
const notReady = [];
// Find each player's ready state
let sql = `SELECT playerTag, readyState FROM room_system_ready_checks WHERE gameId=?`;
const players = await dbQueryAll(sql, [roomSystem.gameId]);
players.forEach((player) => {
if (parseInt(player.readyState, 10) === 1) {
ready.push(player.playerTag);
} else {
notReady.push(player.playerTag);
}
});
const output = ['**Ready:**'];
ready.length === 0 ?
output.push('🏜 No players are ready yet.') :
ready.forEach((player) => output.push(player));
output.push('');// Add a blank line between ready and not-ready players
output.push('**Not ready:**')
notReady.length === 0 ?
output.push('🏁 All players are ready!') :
notReady.forEach((player) => output.push(player));
return message.channel.send(output);
// Lock a dynamic voice channel, preventing anyone except moderators from joining
case '.lock':
return message.guild.channels.resolve(roomSystem.voiceChannelId).overwritePermissions([
{
// @everyone may not join the voice channel
id: message.guild.id,
deny: [ 'CONNECT' ],
},
{
// Moderators should still have full access
id: getModeratorRole(message.guild).id,
allow: [ 'CONNECT' ],
},
{
// @AginahBot should be able to connect
id: client.user.id,
allow: [ 'CONNECT' ],
}
]);
// Reopen a dynamic voice channel, allowing anyone to join
case '.unlock':
return message.guild.channels.resolve(roomSystem.voiceChannelId).overwritePermissions([]);
case '.close':
let voiceChannel = message.guild.channels.resolve(roomSystem.voiceChannelId);
// Do not re-close an already closed channel
if (voiceChannel.name.search(/ \(Closed\)$/g) > -1) { return; }
message.guild.channels.resolve(roomSystem.planningChannelId)
.send(`${message.channel.name.replace(/\b\w/g, c => c.toUpperCase())} is now closed.`);
return voiceChannel.edit({ name: `${voiceChannel.name.toString()} (Closed)` });
}
};<file_sep>/voiceStateListeners/gameActivity.js
const { generalErrorHandler } = require('../errorHandlers');
const { getModeratorRole, dbQueryOne, dbExecute } = require('../lib');
const channelNames = ['Zucchini', 'Artichoke', 'Pineapple', 'Kumquat', 'Avocado', 'Blueberry', 'Mango', 'Strawberry',
'Durian', 'Watermelon', 'Papaya', 'Cherry', 'Nectarine', 'Raspberry', 'Cantaloupe', 'Potato', 'Tomato', 'Broccoli',
'Cauliflower', 'Cucumber', 'Asparagus', 'Rhubarb', 'Eggplant', 'Plantain', 'Banana', 'Apple'];
const randInRange = (min, max) => {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min + 1) + min);
};
module.exports = async (client, oldState, newState) => {
// If the user changed their voice state but remained in the same channel, do nothing (mute, deafen, etc.)
if (oldState.channel && newState.channel && oldState.channel.id === newState.channel.id) { return; }
if (newState.channel) {
// If a user has entered the "Start Game" channel
let sql = `SELECT rs.id, rs.channelCategoryId
FROM room_systems rs
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE gd.guildId=?
AND rs.newGameChannelId=?`;
let roomSystem = await dbQueryOne(sql, [newState.guild.id, newState.channel.id]);
if (roomSystem) {
const channelName = channelNames[randInRange(0, channelNames.length - 1)];
return newState.guild.roles.create({ data: { name: channelName, mentionable: true }}).then((role) => {
Promise.all([
// Voice channel
newState.guild.channels.create(channelName, {
type: 'voice',
parent: roomSystem.channelCategoryId,
}),
// Text channel
newState.guild.channels.create(channelName, {
type: 'text',
parent: roomSystem.channelCategoryId,
permissionOverwrites: [
{
// @everyone may not view the text channel
id: newState.guild.id,
deny: [ 'VIEW_CHANNEL' ],
},
{
// Moderators should be able to view this channel
id: getModeratorRole(newState.guild).id,
allow: [ 'VIEW_CHANNEL' ],
},
{
// @AginahBot may view the text channel
id: client.user.id,
allow: [ 'VIEW_CHANNEL' ],
},
{
// Role assignees may view the channel
id: role.id,
allow: [ 'VIEW_CHANNEL' ],
}
],
})
]).then(async (channels) => {
channels[1].send(`Hello! Use this channel to discuss the ${channelName} game.\n` +
'__Ready Checks:__\n' +
'`.ready` to show you are ready to begin\n' +
'`.unready` to change your mind\n' +
'`.readycheck` to see who is ready\n\n' +
'__Privacy Controls:__\n' +
'`.close` to close this channel\n' +
'`.lock` to prevent others from joining\n' +
'`.unlock` to allow people to join again');
let sql = `INSERT INTO room_system_games (roomSystemId, voiceChannelId, textChannelId, roleId)
VALUES (?, ?, ?, ?)`;
await dbExecute(sql, [roomSystem.id, channels[0].id, channels[1].id, role.id]);
await newState.member.voice.setChannel(channels[0]);
await newState.member.roles.add(role);
}).catch((error) => generalErrorHandler(error));
});
}
// If the user has entered a game channel
sql = `SELECT rs.id, rs.channelCategoryId
FROM room_system_games rsg
JOIN room_systems rs ON rsg.roomSystemId = rs.id
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE rsg.voiceChannelId=?
AND gd.guildId=?`;
roomSystem = await dbQueryOne(sql, [newState.channel.id, newState.guild.id]);
if (roomSystem) {
sql = `SELECT id, roleId FROM room_system_games WHERE roomSystemId=? AND voiceChannelId=?`;
const gameData = await dbQueryOne(sql, [roomSystem.id, newState.channel.id]);
// If the voice channel the user entered is not a game channel, do nothing
if (!gameData) { return; }
// Grant the user the channel role
const role = newState.guild.roles.resolve(gameData.roleId);
newState.member.roles.add(role);
// Add the user to the ready checks table
sql = `INSERT INTO room_system_ready_checks (gameId, playerId, playerTag) VALUES (?,?,?)`;
await dbExecute(sql, [gameData.id, newState.member.id, newState.member.user.tag]);
}
}
if (oldState.channel) {
// User leaves a game channel
let sql = `SELECT rs.id, rs.channelCategoryId
FROM room_system_games rsg
JOIN room_systems rs ON rsg.roomSystemId = rs.id
JOIN guild_data gd ON rs.guildDataId = gd.id
WHERE rsg.voiceChannelId=?
AND gd.guildId=?`;
const roomSystem = await dbQueryOne(sql, [oldState.channel.id, oldState.guild.id]);
if (roomSystem) {
sql = `SELECT id, roleId, textChannelId, voiceChannelId
FROM room_system_games
WHERE roomSystemId=?
AND voiceChannelId=?`;
const channelData = await dbQueryOne(sql, [roomSystem.id, oldState.channel.id]);
// If the voice channel the user left was not a game channel, do nothing
if (!channelData) { return; }
// Remove channel role from this user
const role = oldState.guild.roles.resolve(channelData.roleId);
oldState.member.roles.remove(role);
// Remove user from ready_checks table
sql = `SELECT id FROM room_system_games WHERE voiceChannelId=? AND roomSystemId=?`;
const game = await dbQueryOne(sql, [oldState.channel.id, roomSystem.id]);
sql = `DELETE FROM room_system_ready_checks WHERE gameId=? AND playerId=?`;
await dbExecute(sql, [game.id, oldState.member.id]);
// If the voice channel is now empty, destroy the role and channels
if (oldState.channel.members.array().length === 0) {
role.delete();
oldState.guild.channels.resolve(channelData.textChannelId).delete();
oldState.guild.channels.resolve(channelData.voiceChannelId).delete();
// Delete the database entry for for this channel
await dbExecute(`DELETE FROM room_system_games WHERE id=?`, [channelData.id]);
}
}
}
};<file_sep>/messageListeners/gameHosted.js
const { supportedGames } = require('../assets/supportedGames.json');
const { dbQueryOne } = require('../lib');
module.exports = async (client, message) => {
const watchedUrls = [];
Object.keys(supportedGames).forEach((game) => {
watchedUrls.push(supportedGames[game].seedDownloadUrl);
});
for (let url of watchedUrls) {
if (message.content.search(url) > -1) {
// Ping the channel role to alert the seed has been generated
const sql = `SELECT rsg.roleId AS roleId
FROM room_systems rs
JOIN room_system_games rsg ON rs.id = rsg.roomSystemId
JOIN guild_data gd ON rs.guildDataId=gd.id
WHERE gd.guildId=?
AND rsg.textChannelId=?`;
const roleData = await dbQueryOne(sql, [message.guild.id, message.channel.id]);
if (roleData) {
message.channel.send(`${message.guild.roles.resolve(roleData.roleId)}: The seeds have been posted!`);
// Pin the message to the channel
return message.pin();
}
}
}
}; | d7641a10b3289f03a4756b0a993181682db8a125 | [
"JavaScript",
"Markdown"
] | 11 | JavaScript | zig-for/AginahBot | 505b40067e0f63cc73b32c8d9aa5abb4231cb724 | df62cf398e3db31afe51b8c0efa9efc3c804795c |
refs/heads/master | <file_sep>import requests
import json
import sys
base_url = 'https://pricing.us-east-1.amazonaws.com'
r = requests.get(base_url+"/offers/v1.0/aws/AmazonRDS/current/region_index.json")
json_reponse = r.json()
json_regions = json_reponse['regions']
af_south_1_json = json_regions['af-south-1']
af_south_1_url = af_south_1_json['currentVersionUrl']
r = requests.get(base_url+af_south_1_url)
print(r.text) | 2f553a3bbd11e46dc83c8e7027421faba6ff4cb4 | [
"Python"
] | 1 | Python | jorotenev/DevopsCourseTasks | ac93027ebdb669b3688dab077938f52925087c40 | 564b041718d1613a0f61692d1388a69487ed908c |
refs/heads/master | <repo_name>jsmartini/homeworkHelper<file_sep>/README.md
# homeworkHelper
scripts and programs to streamline engineering homework problems
<file_sep>/vector_op.py
import math
def dcos(x):
return math.cos(math.radians(x))
def dsin(x):
return math.sin(math.radians(x))
def dtan(x):
return math.tan(math.radians(x))
def dot(x , y):
sum = 0
for x, y in zip(x, y):
sum += x*y
return sum
def mag(x):
sum = 0
for i in x:
sum += i**2
return sum**0.5
def cosDot(x, y):
a = dot(x,y)
return a/(mag(x)*mag(y))
def cross(x, y):
return (x[1]*y[2]-y[1]*x[2],-1*(x[0]*y[2]-y[0]*x[2]), x[0]*y[1]-y[0]*x[1])
def sinCross(x,y):
a = mag(cross(x,y))
return a/(mag(x)*mag(y))
def angle(x,y):
return math.degrees(math.acos(cosDot(x,y)))
def add(x, y):
resultant = []
for i, j in zip(x,y):
resultant.append(i + j)
return resultant
def sub(x,y):
return add(x, [i * -1 for i in y])
def mult(a, scalar):
z = []
for f in a:
z.append(f * scalar)
return z
def divide(a, scalar):
z = []
for f in a:
z.append(f/scalar)
return z
def fvec_from_unit(vec, force):
vec = divide(vec, mag(vec))
return mult(vec, force)
def unitv(vec):
return divide(vec, mag(vec))
| e7a25d43d8de65ecfb9612f7bab8aa63f7ddf40b | [
"Markdown",
"Python"
] | 2 | Markdown | jsmartini/homeworkHelper | 3a51dc3019616553e274f29dca14412ee2cd6955 | b6f6b4252cd17408e27aa126816d653bd42c4a10 |
refs/heads/master | <file_sep>var path = require('path');
global.post_uploads_dir = path.resolve(__dirname+"/uploads");
const cors = require('cors');
const express = require('express');
const app = express();
//Routes
const userRoutes = require('./routes/users');
const postRoutes = require('./routes/posts');
require('./config/sequelize');
// Habilitando o Cors
app.use(cors());
// Criar uma codificação padrão para as URL (Sanitizar URL's)
app.use(express.urlencoded({
extended: true
}));
// Conseguir ler o corpo das requisições
app.use(express.json());
app.use('/user', userRoutes);
app.use('/post', postRoutes);
app.listen(3333, function() {
console.log('Server is running')
})<file_sep>const jwt = require('jsonwebtoken');
const crypto = require('crypto');
const { User } = require('../../models');
const create = async (request, response) => {
try {
const { email, password, firstName, lastName } = request.body;
const user = await User.findOne({
where: { email },
});
if (user) {
throw new Error('Usuário já existe');
}
const encryptedPassword = crypto.createHash('sha256').update(password).digest('hex');
const doc_content = {
email,
firstName,
lastName,
password: encryptedPassword,
};
const newUser = await User.create(doc_content);
delete newUser.dataValues.password
return response.status(201).json(newUser)
} catch (error) {
return response.status(403).json({ error: true, errorMessage: error.message })
}
};
const login = async (request, response) => {
try {
function sleep() {
return new Promise(function (resolve) {
setTimeout(resolve, 1000)
})
}
await sleep();
const user = await User.findOne({
where: { email: request.body.email },
});
if (!!user === false) {
throw new Error('Senha ou email incorreto');
}
const reqPass = crypto.createHash('sha256').update(request.body.password || '').digest('hex');
if (reqPass !== user.password) {
throw new Error('Senha ou email incorreto');
}
const token = jwt.sign({
user: {
userId: user.id,
email: user.email,
createdAt: new Date(),
},
},
'123456', //<--------CHAVE SECRETA PARA CRIAR O TOKEN
);
delete user.dataValues.password;
return response.status(200).json({ user, token });
} catch (error) {
console.log('Error => ', error)
return response.status(403).json({ error: true, errorMessage: error.message });
}
};
const validToken = async (request, response) => {
try {
const { token } = request.body;
jwt.verify(token, '<PASSWORD>', (err, decoded) => {
if (!err) {
return response.status(200).json({
token: token,
user: decoded.user,
valid: true
})
} else {
console.log('Token Error err => ', err)
return response.status(403).json({ error: true, errorMessage: err.message })
}
})
} catch (error) {
}
}
module.exports = { create, login, validToken }<file_sep>const db = {
development: {
username: 'gfdoayju',
password: '<PASSWORD>',
database: 'gfdoayju',
host: 'tuffi.db.elephantsql.com',
dialect: 'postgres',
}
};
module.exports = db;
<file_sep>const express = require('express');
const userController = require('../controllers/user/user.controller');
const router = express.Router();
const { valid_login } = require('../validations/user')
//= ===============================
// User routes
//= ===============================
router.post(
'/',
userController.create,
);
router.post(
'/validate_token',
userController.validToken,
);
router.post(
'/login',
valid_login,
userController.login,
);
module.exports = router;
<file_sep>const fs = require('fs');
const { Posts } = require('../../models')
const create = async (request, response) => {
const json_info = JSON.parse(request.body.info);
const createdPost = await Posts.create({
title: json_info.title,
description: json_info.description,
uri: `http://localhost:3333/post/${request.file.filename}`
})
// ...
return response.json(createdPost)
};
const read = (request, response) => {
const filePath = post_uploads_dir + "/" + request.params.audio_id;
var stat = fs.statSync(filePath);
var total = stat.size;
if (request.headers.range) {
var range = request.headers.range;
var parts = range.replace(/bytes=/, "").split("-");
var partialstart = parts[0];
var partialend = parts[1];
var start = parseInt(partialstart, 10);
var end = partialend ? parseInt(partialend, 10) : total - 1;
var chunksize = (end - start) + 1;
var readStream = fs.createReadStream(filePath, { start: start, end: end });
response.writeHead(206, {
'Content-Range': 'bytes ' + start + '-' + end + '/' + total,
'Accept-Ranges': 'bytes', 'Content-Length': chunksize,
'Content-Type': 'audio/mp3'
});
readStream.pipe(response);
} else {
response.writeHead(200, { 'Content-Length': total, 'Content-Type': 'audio/mp3' });
fs.createReadStream(filePath).pipe(response);
}
};
const list = async (request, response) => {
try {
const posts = await Posts.findAll();
return response.json(posts)
} catch (error) {
console.log('Posts List => ', error)
return response.status(500).json({error: true, errorMessage: error.message})
}
}
module.exports = { create, read, list }<file_sep>const Sequelize = require('sequelize');
const config = require('../config/database').development;
const db = {};
const models = [
require('../models/user'),
require('../models/posts'),
]
const sequelize = new Sequelize(
config.database,
config.username,
config.password,
config,
);
/**
* db = {
* User: ...
* }
*/
models.forEach((notInitializedModel) => {
const model = notInitializedModel(sequelize, Sequelize.DataTypes)
db[model.name] = model;
if(db[model.name].associate) {
db[model.name].associate(db);
}
});
db.sequelize = sequelize;
db.Sequelize = Sequelize;
module.exports = db;
<file_sep>const valid_login = (req, resp, next) => {
const { email, password } = req.body;
try {
if (email && password && typeof(email) === 'string' && typeof(password) === 'string') {
console.log('Login Middleware');
next();
} else {
throw new Error('Senha ou Email incorretos')
}
} catch (error) {
return resp.status(403).json({ error: true, errorMessage: error.message })
}
}
module.exports = { valid_login } | 3c1f4fb10b9458addebdedd8535c1f2a032e1fcc | [
"JavaScript"
] | 7 | JavaScript | duduneto/taking-cp-node-api | 588cc1e5913b17bc7d5b44c81379a639d6e41369 | 25473ee789283b40ddebfa09df0557adb436dcf4 |
refs/heads/master | <repo_name>myroslavHub/CommentsApp<file_sep>/commentsapi/Controllers/CommentsController.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
using Microsoft.EntityFrameworkCore;
using commentsapi.Models;
namespace commentsapi.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class CommentsController : ControllerBase
{
private readonly CommentsContext _context;
public CommentsController(CommentsContext context)
{
_context = context;
}
// GET: api/Comments/5
[HttpGet("{id}")]
public async Task<ActionResult<Comment>> GetComment(int id)
{
var comment = await _context.Comments.FindAsync(id);
if (comment == null)
{
return NotFound();
}
return comment;
}
// PUT: api/Comments/5
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see https://aka.ms/RazorPagesCRUD.
[HttpPut("{id}")]
public async Task<IActionResult> PutComment(int id, Comment comment)
{
if (id != comment.Id)
{
return BadRequest();
}
_context.Entry(comment).State = EntityState.Modified;
try
{
await _context.SaveChangesAsync();
}
catch (DbUpdateConcurrencyException)
{
if (!CommentExists(id))
{
return NotFound();
}
else
{
throw;
}
}
return CreatedAtAction("GetComment", new { id = comment.Id }, comment);
}
[HttpPut("update/{id}")]
public async Task<IActionResult> UpdateComment(int id, Comment commentToUpdate)
{
var comment = await _context.Comments.FindAsync(id);
if (comment == null)
{
return NotFound();
}
comment.Text = commentToUpdate.Text;
_context.Entry(comment).State = EntityState.Modified;
try
{
await _context.SaveChangesAsync();
}
catch (DbUpdateConcurrencyException)
{
if (!CommentExists(id))
{
return NotFound();
}
else
{
throw;
}
}
return CreatedAtAction("GetComment", new { id = comment.Id }, comment);
}
[HttpPut("like/{id}")]
public async Task<IActionResult> LikeComment(int id)
{
var comment = await _context.Comments.FindAsync(id);
if (comment == null)
{
return NotFound();
}
comment.Likes++;
_context.Entry(comment).State = EntityState.Modified;
try
{
await _context.SaveChangesAsync();
}
catch (DbUpdateConcurrencyException)
{
if (!CommentExists(id))
{
return NotFound();
}
else
{
throw;
}
}
return CreatedAtAction("GetComment", new { id = comment.Id }, comment);
}
[HttpPut("dislike/{id}")]
public async Task<IActionResult> DislikeComment(int id)
{
var comment = await _context.Comments.FindAsync(id);
if (comment == null)
{
return NotFound();
}
comment.Likes--;
_context.Entry(comment).State = EntityState.Modified;
try
{
await _context.SaveChangesAsync();
}
catch (DbUpdateConcurrencyException)
{
if (!CommentExists(id))
{
return NotFound();
}
else
{
throw;
}
}
return CreatedAtAction("GetComment", new { id = comment.Id }, comment);
}
[HttpPost("{parentCommentId}")]
public async Task<ActionResult<Comment>> PostComment(int parentCommentId, Comment comment)
{
var t = await _context.Comments.FindAsync(parentCommentId);
t.Comments.Add(comment);
await _context.SaveChangesAsync();
return CreatedAtAction("GetComment", new { id = comment.Id }, comment);
}
[HttpPost("addfortopic/{topicId}")]
public async Task<ActionResult<Comment>> PostCommentForTopic(int topicId, Comment comment)
{
var t = await _context.Topics.Include(t=>t.Comments).FirstAsync(t=>t.Id == topicId);
t.Comments.Add(comment);
await _context.SaveChangesAsync();
return CreatedAtAction("GetComment", new { id = comment.Id }, comment);
}
// DELETE: api/Comments/5
[HttpDelete("{id}")]
public async Task<ActionResult<Comment>> DeleteComment(int id)
{
var comment = await _context.Comments.FindAsync(id);
if (comment == null)
{
return NotFound();
}
_context.Comments.Remove(comment);
await _context.SaveChangesAsync();
return comment;
}
private bool CommentExists(int id)
{
return _context.Comments.Any(e => e.Id == id);
}
}
}
<file_sep>/commentsapi/Models/Topic.cs
using System;
using System.Collections.Generic;
namespace commentsapi.Models
{
public class Topic
{
public Topic()
{
Comments = new List<Comment>();
}
public long Id { get; set; }
public string Author { get; set; }
public string Name { get; set; }
public string Description { get; set; }
public DateTime Date { get; set; }
public ICollection<Comment> Comments { get; set; }
}
}<file_sep>/commentsapi/Dto/TopicDto.cs
using System;
namespace commentsapi.Dto
{
public class TopicDto
{
public TopicDto()
{
}
public long Id { get; set; }
public string Author { get; set; }
public string Name { get; set; }
public string Description { get; set; }
public int CommentsCount{ get; set; }
public DateTime Date { get; set; }
}
}<file_sep>/commentsapi/Controllers/TopicsController.cs
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
using Microsoft.EntityFrameworkCore;
using commentsapi.Models;
using commentsapi.Dto;
namespace commentsapi.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class TopicsController : ControllerBase
{
private readonly CommentsContext _context;
public TopicsController(CommentsContext context)
{
_context = context;
}
[HttpGet]
public async Task<ActionResult<IEnumerable<TopicDto>>> GetTopics()
{
var topics = await _context.Topics.Include(t => t.Comments).ToListAsync();
var comments = await _context.Comments.ToListAsync();
return topics.Select(topic=>new TopicDto(){
Id = topic.Id,
Author = topic.Author,
Name = topic.Name,
Description = topic.Description,
Date = topic.Date,
CommentsCount = comments.Where(com => topic.Comments.Any(c => c.Id == com.Id)).ToList().Select(GetCommentsCount).Sum()
}).ToList();
}
private int GetCommentsCount(Comment comment){
if (comment.Comments == null || comment.Comments.Count==0){
return 1;
}
return comment.Comments.Select(GetCommentsCount).Sum() + 1;
}
[HttpGet("{topicId}/comments")]
public async Task<ActionResult<IEnumerable<Comment>>> GetCommentsForTopic(int topicId)
{
var topic = await _context.Topics.Include(t => t.Comments).FirstAsync(t => t.Id == topicId);
var comments = await _context.Comments.ToListAsync();
return comments.Where(com => topic.Comments.Any(c => c.Id == com.Id)).ToList();
}
// GET: api/Topics/5
[HttpGet("{id}")]
public async Task<ActionResult<Topic>> GetTopic(long id)
{
var topic = await _context.Topics.Include(t => t.Comments).ThenInclude(c => c.Comments).FirstAsync(t => t.Id == id); // .FindAsync(id);
//var topic = await _context.Topics.FindAsync(id);
if (topic == null)
{
return NotFound();
}
return topic;
}
// PUT: api/Topics/5
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see https://aka.ms/RazorPagesCRUD.
[HttpPut("{id}")]
public async Task<IActionResult> PutTopic(long id, Topic topic)
{
if (id != topic.Id)
{
return BadRequest();
}
_context.Entry(topic).State = EntityState.Modified;
try
{
await _context.SaveChangesAsync();
}
catch (DbUpdateConcurrencyException)
{
if (!TopicExists(id))
{
return NotFound();
}
else
{
throw;
}
}
return NoContent();
}
// POST: api/Topics
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see https://aka.ms/RazorPagesCRUD.
[HttpPost]
public async Task<ActionResult<Topic>> PostTopic(Topic topic)
{
_context.Topics.Add(topic);
await _context.SaveChangesAsync();
return CreatedAtAction("GetTopic", new { id = topic.Id }, topic);
}
// DELETE: api/Topics/5
[HttpDelete("{id}")]
public async Task<ActionResult<Topic>> DeleteTopic(long id)
{
var topic = await _context.Topics.FindAsync(id);
if (topic == null)
{
return NotFound();
}
_context.Topics.Remove(topic);
await _context.SaveChangesAsync();
return topic;
}
private bool TopicExists(long id)
{
return _context.Topics.Any(e => e.Id == id);
}
}
}
<file_sep>/commentsapi/Models/Context.cs
using Microsoft.EntityFrameworkCore;
namespace commentsapi.Models
{
public class CommentsContext : DbContext
{
public DbSet<Topic> Topics { get; set; }
public DbSet<Comment> Comments { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder.UseSqlite(@"Data Source=CommentsDB.db;");
}
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Comment>()
.HasMany(c => c.Comments)
.WithOne()
.HasForeignKey("ParentId").IsRequired(false).OnDelete(DeleteBehavior.Cascade);
//modelBuilder.Entity<Comment>().HasOne(c => c.Parent).WithMany(c => c.Comments).OnDelete(DeleteBehavior.Cascade);
modelBuilder.Entity<Topic>()
.HasMany(c => c.Comments)
.WithOne().HasForeignKey("TopicId").IsRequired(false).OnDelete(DeleteBehavior.Cascade);
}
}
}<file_sep>/README.md
# CommentsApp
#### API написано на .net core.
Знаходиться за цією адресою: http://commentsapi.azurewebsites.net/
Документацію по API методах можна найти тутка: http://commentsapi.azurewebsites.net/swagger/index.html
#### Веб частина створена на Flutter Web.
Знаходиться за цією адресою: http://commentsui.azurewebsites.net/
При вході буде запропоновано ввести ім'я. Після чого, відбудеться перехід на сторінку привітання.

Ім'я зберігається, і при наступних відвіданах немає необхідності знову вводити ім'я.

Припереході далі, буде відображено список тем для обговорення. Для кожної теми вказано автора, кількість коментарів, та можливість її видалити, якщо її було створеним активним користувачем.

Знизу списку є кнопка для додавання нової теми, при натисканні відкриється діалог.

У верхній частині розташоване лого, та імя користувача. Його можна змінити нажавши на нього.

Нажавши на тему, відбудеться перехід на наступний екран. На ньому відображаються коментарі до теми, і до інших коментарів. Автор коментаря має можливість змінити його чи видалити. Будь хто може лайкати та створювати нові коментарі під існуючими.



<file_sep>/commentsapi/Models/Comment.cs
using System;
using System.Collections.Generic;
namespace commentsapi.Models
{
public class Comment
{
public Comment()
{
Comments = new List<Comment>();
}
public int Id { get; set; }
public int Likes { get; set; }
public string Text { get; set; }
public string Author { get; set; }
public DateTime Date { get; set; }
public ICollection<Comment> Comments { get; set; }
}
} | 146f1d2311662579bef40bfcf475c6d72baef8a5 | [
"Markdown",
"C#"
] | 7 | C# | myroslavHub/CommentsApp | b86632a7a572ceeef61840bc6cf66dab015d1fe2 | 15ab9691f7f5d613d9faadc32bb5a4d907ed6570 |
refs/heads/master | <repo_name>moChen0607/curveDeformer<file_sep>/src/vtCurveDeformer.py
import maya.OpenMaya as om
import maya.OpenMayaMPx as omMpx
import maya.OpenMayaAnim as omAnim
import maya.OpenMayaRender as OpenMayaRender
import maya.OpenMayaUI as OpenMayaUI
import sys
import numpy as np
from math import sqrt
from random import randint
#sys.path.insert(0, '/Users/fruity/Documents/_dev/fToolbox/vtPlugins/vtCurveDeformer/src/')
import nurbsCurve;reload(nurbsCurve)
pluginName = 'curveDeformer'
pluginId = om.MTypeId(0x1272C9)
glRenderer = OpenMayaRender.MHardwareRenderer.theRenderer()
glFT = glRenderer.glFunctionTable()
np.set_printoptions(precision=3)
'''
cmds.delete(df)
cmds.flushUndo()
cmds.unloadPlugin('vtCurveDeformer')
cmds.file('/Users/fruity/Documents/_dev/curveDeformer/scenes/example_scene_2015.ma', o=1, f=1)
cmds.loadPlugin('/Users/fruity/Documents/_dev/fToolbox/vtPlugins/vtCurveDeformer/src/vtCurveDeformer.py')
df = cmds.deformer('pCylinder1', type='curveDeformer')[0]
cmds.connectAttr('inCrv.worldSpace', df + '.inCrv')
cmds.connectAttr('baseCrv.worldSpace', df + '.baseCrv')
cmds.connectAttr('P.worldMatrix', df + '.matrixJoints[0].matrixJoint')
cmds.connectAttr('O.worldMatrix', df + '.matrixJoints[1].matrixJoint')
cmds.connectAttr('Q.worldMatrix', df + '.matrixJoints[2].matrixJoint')
cmds.refresh()
cmds.setAttr(df + '.initialize', False)
'''
class curveDeformer(omMpx.MPxDeformerNode):
'''
From what I understood, we have roughly 5 steps:
- build a matrix for each CV. Pos is the pos of the CV, and rotations are
computed using joint orientations (if both jointA and jointB has a weight
of .5, the matrix of the CV will have an orientation matrix based on
jointA/jointB slerp-ed at .5)
- for each vertex, offset each CV of the inCrv by delta, which is the
vector [closest point from the vtx on the curve -> vtx]. Each CV being
offseted, we have a new -offset- curve passing through the vertex
- moves individually each CV of the offset curves, based on :
1 - the angle of the bones (in the paper, shoulder = P, elbow = O,
wrist = Q, and the current vertex = R). This multiplier,
computed in Eq. 11, is known as Tau
2 - another multiplier, based on the distance from the vertex to the
mid joint (i.e. O). This is, in the MOS paper (dreamworks), detailled
as the "inverse distance weighting" strategy
- knowing the direction in which we need to push (or pull) the CV, we
multiply this direction by Tau and the CV weight
- finally, we get the point at parameter for the current vtx, on the fix crv
'''
aInit = om.MObject()
aInCrv = om.MObject()
aBaseCrv = om.MObject()
aWeight = om.MObject()
aCps = om.MObject()
aMatrixJoint = om.MObject()
aMatrixJoints = om.MObject()
if om.MGlobal.apiVersion() < 201600:
_input = omMpx.cvar.MPxDeformerNode_input # note: input is a python builtin
inputGeom = omMpx.cvar.MPxDeformerNode_inputGeom
envelope = omMpx.cvar.MPxDeformerNode_envelope
outputGeom = omMpx.cvar.MPxDeformerNode_outputGeom
else:
_input = omMpx.cvar.MPxGeometryFilter_input # note: input is a python builtin
inputGeom = omMpx.cvar.MPxGeometryFilter_inputGeom
envelope = omMpx.cvar.MPxGeometryFilter_envelope
outputGeom = omMpx.cvar.MPxGeometryFilter_outputGeom
def __init__(self):
omMpx.MPxDeformerNode.__init__(self)
def deform(self, data, itGeo, localToWorldMatrix, geomIndex):
#
# get input datas
hDeformedMeshArray = data.outputArrayValue(curveDeformer._input)
hDeformedMeshArray.jumpToElement(geomIndex)
hDeformedMeshElement = hDeformedMeshArray.outputValue()
oDeformedMesh = hDeformedMeshElement.child(curveDeformer.inputGeom).asMesh()
fnDeformedMesh = om.MFnMesh(oDeformedMesh)
# ----------------------------------------------------------------------
# GET THE ATTRIBUTES
# ----------------------------------------------------------------------
# get the init state
initialize = data.inputValue(self.aInit).asBool()
# get the in curve
oCrv = data.inputValue(curveDeformer.aInCrv).asNurbsCurve()
if oCrv.isNull(): return
fnCrv = om.MFnNurbsCurve(oCrv)
# get the base curve
oBaseCrv = data.inputValue(self.aBaseCrv).asNurbsCurve()
if oBaseCrv.isNull(): return
fnBaseCrv = om.MFnNurbsCurve(oBaseCrv)
# get general curve infos
degree = fnCrv.degree()
dummy = om.MDoubleArray()
fnCrv.getKnots(dummy)
knots = [dummy[i] for i in xrange(dummy.length())]
knots = [knots[0]] + knots + [knots[-1]]
# envelope
envelopeHandle = data.inputValue(curveDeformer.envelope)
env = envelopeHandle.asFloat()
if not env: return
# get the matrix of each joint (to compute Tau)
self.jts_pos = []
hMatrixJointsArray = data.inputArrayValue(self.aMatrixJoints)
if hMatrixJointsArray.elementCount() < 3:
return # we need at least 3 joints to compute Tau
for i in xrange(hMatrixJointsArray.elementCount()):
hMatrixJointsArray.jumpToArrayElement(i)
jt_mat = om.MTransformationMatrix(hMatrixJointsArray.inputValue().child(curveDeformer.aMatrixJoint).asMatrix())
vPos = jt_mat.getTranslation(om.MSpace.kWorld)
self.jts_pos.append([vPos.x, vPos.y, vPos.z])
# get the control points and the weights
weights = [] # list of floats
hCvArray = data.inputArrayValue(self.aCps)
for i in xrange(hCvArray.elementCount()):
hCvArray.jumpToArrayElement(i)
fWeight = hCvArray.inputValue().child(curveDeformer.aWeight).asFloat()
weights.append(fWeight)
# ----------------------------------------------------------------------
# get the CVs of both the base and normal curves
cvs_array = om.MPointArray()
cvs_base_array = om.MPointArray()
fnCrv.getCVs(cvs_array)
fnBaseCrv.getCVs(cvs_base_array)
# make sure the weights array have a valid length (as many elements as there are CVs)
num_cvs = cvs_array.length()
if len(weights) < num_cvs:
weights.extend([1] * (num_cvs - len(weights)))
elif len(weights) > num_cvs:
weights = weights[:num_cvs]
# ----------------------------------------------------------------------
# INITIALIZE
# ----------------------------------------------------------------------
# at init stage, we do :
# 1 - get the skinCluster of the curve
# 2 - get the matrices / weights of the joints influencing the SC
# 3 - get an average matrix for each CP
# 4 - get the offset vector delta between closest point on curve
# and current vertex
# 5 - get the 3 closest joints for each vertex and compute Tau
# 6 - assign a weight for each offset CV based on the distance with the vtx
if initialize:
# 1 - get the skinCluster attached to the curve and the dag path
fnSc, dpInCrv = self.get_skin_cluster()
# 2 - get the bones and weights
self._weights = self.get_skin_weights(fnSc, dpInCrv)
# 3 - compute the base transformation matrix for each CV
self._dpJoints = om.MDagPathArray()
fnSc.influenceObjects(self._dpJoints)
self._base_mats_per_cv = self.get_mat_per_cv(self._dpJoints, cvs_base_array)
# 4 - compute offset vector and parameter
self._pOffsets, self._params = self.get_offsets_and_params(itGeo, fnBaseCrv)
# 5 - get the 3 closest joints for each vertex, to compute Tau parameter later. This is a list of the 3 closest joint indices, for each vertex
self._closest_jts_idx = self.get_3_closest_jts_per_vertex(itGeo, self.jts_pos)
P, O, Q = self._closest_jts_idx[itGeo.index()]
# 6 - assign a weight to each offset cv, based on inv dist from O
self._dist_CV_weights = self.inverse_distance_weighting(O, cvs_base_array)
# 7 - set the direction of the offset CVs method (in deform)
self._directions_mat = self.set_offset_direction(itGeo, self._pOffsets, cvs_base_array, self._base_mats_per_cv)
# 8 - get the Tau values by default to remap them efficiently later
self._default_taus = self.get_default_taus(itGeo, P, O, Q)
# ----------------------------------------------------------------------
# DEFORM
# ----------------------------------------------------------------------
# to rebuild the curve, we need to get 2 things :
# - the offset between the current vertex and the closest point
# on curve computed in the initialize and stored in self._pOffsets
# - the transformationMatrix between all the CVs of the base_crv and the crv
# once we have that, we just add the offset to the transformMatrix to get the
# virtual cvs of the offset curve
else:
out_positions = om.MPointArray()
while not itGeo.isDone():
# compute the Tau multiplier
P, O, Q = self._closest_jts_idx[itGeo.index()]
offset_cvs = []
weighted_matrices = []
# self._np_jts_pos()
for i in xrange(cvs_array.length()):
# ----------------------------------------
# get the weighted matrix
euler_per_joint = []
for j in xrange(self._dpJoints.length()):
inclusive_mat = self._dpJoints[j].inclusiveMatrix()
euler_per_joint.append(om.MTransformationMatrix(inclusive_mat).rotation().asEulerRotation())
# - get the offset matrix (cv * base_cv. The baseCV mat has
# a position 0,0,0, so with only 1 matrix mult, we get the
# offset in the correct position in space instead of
# having it in the origin
weighted_matrix = self.get_weighted_matrix(euler_per_joint, self._weights[i], cvs_array[i])
weighted_base_matrix = self._base_mats_per_cv[i]
offset_mat = weighted_matrix * weighted_base_matrix.inverse()
weighted_matrices.append(weighted_matrix)
# adds the delta
# It is super important to work with MPoints and not
# MVectors for the deltas, as an MPoint*MMatrix gives
# different result from MVector*MMatrix
delta = self._pOffsets[itGeo.index()]
final_pos = delta * offset_mat
# stores the final cv position
offset_cvs.append([final_pos.x, final_pos.y, final_pos.z])
# fix with Tau
# do = 99
# if itGeo.index() == do:
tau = self.get_tau(P, O, Q, om.MPoint(itGeo.position()))
default_tau = self._default_taus[itGeo.index()]
tau = tau - default_tau
# print 'tau -->', tau
# print 'weight -->', self._dist_CV_weights
# tau = self._remap(tau, default_tau-.5, default_tau+.5, -1., 1.)
# get the CVs by distance
# weighted_cvs = self._dist_CV_weights[itGeo.index()]
weighted_cvs = self._dist_CV_weights
offset_cvs = self.offset_CVs_by_tau(itGeo.position(),
offset_cvs,
weighted_matrices,
tau,
weighted_cvs,
self._directions_mat[itGeo.index()])
# now we have the new CP positions, compute the curve
crv = nurbsCurve.NurbsCurve(points=offset_cvs, knots=knots, degree=degree, weights=weights)
new_pos = crv.pt_at_param(self._params[itGeo.index()])
out_positions.append(om.MPoint(new_pos[0], new_pos[1], new_pos[2]))
itGeo.next()
itGeo.setAllPositions(out_positions)
def get_skin_cluster(self):
'''
Also returns the dag path to the inCurve, that is needed for
skinCluster.getWeights()
'''
# 1 - get the skinCluster attached to the in curve
# - first, get the plug of the curve
fnDep = om.MFnDependencyNode(self.thisMObject())
pInCrv = fnDep.findPlug(curveDeformer.aInCrv)
itDg = om.MItDependencyGraph(pInCrv, om.MItDependencyGraph.kDownstream, om.MItDependencyGraph.kPlugLevel)
# - then, get the curve as a MDagPath. The reason why we do all this
# crap is because the dataHandle.asNurbsCurve() returns a
# kNurbsCurveData, and MDagPath or everything else requires a kNurbsCurve.
dpInCrv = om.MDagPath()
plugs = om.MPlugArray()
pInCrv.connectedTo(plugs, True, False)
plug = plugs[0]
oInCrv_asNurbsCurve = plug.node()
fnDagInCrv = om.MFnDagNode(oInCrv_asNurbsCurve)
fnDagInCrv.getPath(dpInCrv)
# - now we have this dag path, let's grab the skinCluster
scs = []
while not itDg.isDone():
oCurrent = itDg.currentItem()
if oCurrent.hasFn(om.MFn.kSkinClusterFilter):
scs.append(oCurrent)
itDg.next()
if not scs:
raise TypeError, 'No skin cluster found. Returns'
fnSc = omAnim.MFnSkinCluster(scs[0])
return fnSc, dpInCrv
def get_skin_weights(self, fnSc, dpInCrv):
'''
Returns an array of weights for each CV
:param fnSc: function set of the skinCluster
:type fnSc: MFnSkinCluster
:param dpInCrv: dag path to the curve attached to the skinCluster
:return : list of MDoubleArray
'''
weights = []
itCv = om.MItCurveCV(dpInCrv)
outArray = om.MDoubleArray()
nbInflUtil = om.MScriptUtil()
nbInflUtil.createFromInt(0)
while not itCv.isDone():
outArray.clear()
fnSc.getWeights(dpInCrv, itCv.currentItem(), outArray, nbInflUtil.asUintPtr())
weights.append(list(outArray))
itCv.next()
return weights
def inverse_distance_weighting(self, pt, poses, p=2):
'''
Returns a vector of normalized weights, based on the
inverse distance to the reference point. If one of the
poses is on the reference point (i.e. distance=0),
we can't do the invert 1/0, so we edit manually the
vector to have something like [0,...,0,1,0,...]
Ex : pt = np.zeros([3])
poses = np.array([[-2,0,0],
[-1,0,0],
[0,0,0],
[2,0,0]])
wts = inverse_distance_weighting(o, poses)
:param pt: reference point, that is responsible for the weighing
:type pt: np.array of n elements
:param poses: matrix of shape MxN of each pose we want to assign a weight to
:type poses: np.array of MxN elements
:return: np.array of n elements, representing the weights
'''
num_poses = poses.length()
weights_vec = np.zeros([num_poses])
for i in xrange(num_poses):
cv = np.array([poses[i].x, poses[i].y, poses[i].z])
dist = np.linalg.norm(pt - cv)
inv_dist = 1./ np.power(dist, p) if dist != 0 else -1
weights_vec[i] = inv_dist
if -1 in weights_vec:
for i in xrange(len(weights_vec)):
if weights_vec[i] == -1:
weights_vec[i] = 1
else:
weights_vec[i] = 0
weights_vec = weights_vec / np.sum(weights_vec)
return weights_vec
def get_mat_per_cv(self, dpJoints, cvs_array):
''' Computes an average of the weight for each CV, to build
a single matrix that is the orientation of the current CV.
This matrix is the weighted sum of all the joints that influence
this CV. Right now, it is using euler.
TODO - use quaternions to blend the different matrices
weighted quats implementation :
https://stackoverflow.com/questions/12374087/average-of-multiple-quaternions
:param dpJoints: dag path array for all the joints influencing the curve
:type dpJoints: MDagPathArray
'''
euler_per_joint = []
for j in xrange(dpJoints.length()):
inclusive_mat = dpJoints[j].inclusiveMatrix()
euler_per_joint.append(om.MTransformationMatrix(inclusive_mat).rotation().asEulerRotation())
base_mats_per_cv = om.MMatrixArray()
for i in xrange(cvs_array.length()):
# get the weighted matrix, using euler
transf_mat = self.get_weighted_matrix(euler_per_joint, self._weights[i])#, cvs_array[i])
base_mats_per_cv.append(transf_mat)
return base_mats_per_cv
def get_offsets_and_params(self, itGeo, fnBaseCrv):
'''
Computes the offset between each vertex and the closest point on
the curve. And since it's the same command, compute the param of
the closest point on curve at the same time, to feed the nurbsCurve
algorithm with it
'''
util = om.MScriptUtil()
util.createFromDouble(0.)
uPtr = util.asDoublePtr()
offsets = om.MPointArray()
params = []
all_base_mats = []
while not itGeo.isDone():
# - offset & parameter
pos = itGeo.position()
pt_on_base_crv = fnBaseCrv.closestPoint(pos, uPtr)
param = om.MScriptUtil.getDouble(uPtr)
vOffset = pos - pt_on_base_crv
offsets.append(om.MPoint(vOffset))
params.append(param)
# all_base_mats.append(self._base_mats_per_cv)
itGeo.next()
itGeo.reset()
return offsets, params
def get_weighted_matrix(self, eulers, weights, pos=None):
'''
Takes an array of eulers, an array of weights of the same size,
and outputs one matrix based on the input weights (and the pos)
The rotation is done by interpolating each joint, but the translate is
given (usually the position of the CP)
'''
outX, outY, outZ = 0., 0., 0.
for i in xrange(len(weights)):
curr_euler = eulers[i]
curr_weight = weights[i]
outX += curr_euler.x * curr_weight
outY += curr_euler.y * curr_weight
outZ += curr_euler.z * curr_weight
outEuler = om.MEulerRotation(outX, outY, outZ)
outMatrix = outEuler.asMatrix()
# add the translates if they are provided
if pos:
transf_mat = om.MTransformationMatrix(outMatrix)
transf_mat.setTranslation(om.MVector(pos), om.MSpace.kWorld)
return transf_mat.asMatrix()
else:
return outMatrix
def get_3_closest_jts_per_vertex(self, itGeo, joints_pos):
''' In order to compute Tau, we need to compute the angle
at the elbow, between shoulder and wrist. This is in an ideal setup
with only 3 bones. But if we have more than 3 bones, to do the same
computation, we need to make sure we work on the correct set of 3 bones.
Therefore, we need to check the 3 bones we'll used, regarding the
hierarchy :
Situation 1 - get the closest bone
get its child
get its parent
Situation 2 - get the closest bone
get its child -> NO CHILD
get its parent
get the parent's parent.
Situation 3 - get the closest bone
get its child
get its parent -> NO PARENT
get the child's child.
:param itGeo: iterator for the geometry
:type itGeo: MItGeometry
:param joints_pos: XYZ coordinates for each joint
:type joints_pos: list of np.array
:param joints_hierarchy: list of joints, sorted by parent/child
:type joints_hierarchy: list of np.array
:return : a nested list containing for each vertex a list of 3 indices
:return type : list
'''
# iter through each vertex to keep the 3 closest joints
all_closest_jts_idx = []
while not itGeo.isDone():
pos = itGeo.position()
pos = np.array([pos.x, pos.y, pos.z])
dists = []
for i in xrange(len(joints_pos)):
dists.append(np.linalg.norm(joints_pos[i] - pos))
sorted_dists = sorted(dists)
closest_jt_idx = dists.index(sorted_dists[0])
# - get the child
child_jt_idx = closest_jt_idx+1
# - get the parent
parent_jt_idx = closest_jt_idx-1
# Situation 1
closest_3_jts_idx = [parent_jt_idx, closest_jt_idx, child_jt_idx]
# Situation 2 - no child available
if closest_jt_idx >= len(joints_pos)-1:
closest_3_jts_idx = [parent_jt_idx-1, parent_jt_idx, closest_jt_idx]
# Situation 3 - no parent available
if closest_jt_idx == 0:
closest_3_jts_idx = [closest_jt_idx, child_jt_idx, child_jt_idx+1]
# sorted_dists = sorted_dists[:3]
# closest_3_jts_idx = [dists.index(sorted_dists[0]),
# dists.index(sorted_dists[1]),
# dists.index(sorted_dists[2])]
all_closest_jts_idx.append(closest_3_jts_idx)
itGeo.next()
itGeo.reset()
return all_closest_jts_idx
def get_default_taus(self, itGeo, P, O, Q):
'''
compute the default Tau value for each vertex, in order to know how to
remap it later, in the deform (if we're in the middle of the elbow,
tau may be .5, but on the sides, it may be 1.2. So we need to call a
remap with different values)
'''
out_taus = np.zeros([itGeo.count()])
while not itGeo.isDone():
out_taus[itGeo.index()] = self.get_tau(P, O, Q, itGeo.position())
itGeo.next()
itGeo.reset()
return out_taus
def get_tau(self, p_idx, o_idx, q_idx, vR):
'''
To know how much the CVs need to be offset to sharpen / smooth
the curve, we compute a parameter at bind pause (we call it Tau), that
will vary based on the bend angle between each joint. In this setup,
shoulder, elbow and wrist positions are respectively P, O and Q. The
vertex we currently compute is known as R
:param p_pos: position of the bone P, usually the shoulder
:type p_pos: np.array
:param o_pos: position of the bone P, usually the elbow, which rotates
:type o_pos: np.array
:param q_pos: position of the bone P, usually the wrist
:type q_pos: np.array
:param r_pos: position of the vertex we use to get tau
:type r_pos: np.array
:return : float
'''
p_pos = np.array(self.jts_pos[p_idx])
o_pos = np.array(self.jts_pos[o_idx])
q_pos = np.array(self.jts_pos[q_idx])
r_pos = np.array([vR.x, vR.y, vR.z])
# Eq. 1-3
p = p_pos - o_pos
p_norm = p / np.linalg.norm(p)
q = q_pos - o_pos
q_norm = q / np.linalg.norm(q)
r = r_pos - o_pos
r_norm = r / np.linalg.norm(r)
# Eq. 4-5
theta = np.arccos(r_norm.dot(q_norm)) # angle ROQ en radians
alpha_min = np.arccos(p_norm.dot(q_norm)) # angle POQ en radians
# Eq. 6 - make sure we always have the smaller angle
cross_pq = np.cross(p_norm, q_norm)
cross_rq = np.cross(r_norm, q_norm)
# cross_pq_norm = cross_pq / np.linalg.norm(cross_pq) # pas besoin de normaliser je crois
# cross_rq_norm = cross_rq / np.linalg.norm(cross_rq)
# if cross_pq_norm.dot(cross_rq_norm) >= 0:
if cross_pq.dot(cross_rq) >= 0:
alpha = alpha_min
else:
alpha = 2*np.pi - alpha_min
# alpha est flat quand alpha * (np.pi/alpha) == np.pi
theta_flat = theta * (np.pi/alpha)
# Eq. 9
epsilon = np.linalg.norm(r) * np.cos(theta_flat) # epsilon = distance depuis O jusqu'a la perpidenculaire a OQ en R
# Eq. 10
a = np.linalg.norm(p)
b = np.linalg.norm(q)
numerator = a + a*min(0, epsilon) + b*max(0, epsilon)
# numerator = a + np.linalg.norm(p*min(0, epsilon)) + np.linalg.norm(q*max(0, epsilon))
denominator = a + b
tau = numerator / denominator
return tau
def set_offset_direction(self, itGeo, offsets, base_cvs, base_mat_bones):
'''
In order to know in which direction we'll push the CVs (using Tau) in
the deform, we set a matrix of values (+1 or -1) that we'll
use in offset_CVs_by_tau() later. In a nutshell, we get the vector
offset_CV->vertex, and we compare it against the main orient axis of the
bone (usually -and hardcoded here- +X), and the neg main orient axis
(-X). Then, with cosine similarity, we define if offset_CV-> vertex is
closer from +X or -X.
Returns a m x n matrix with m = number of vertices and n = number of CVs
each value is either 1 or -1, depending if we wanna push or pull the CV
'''
num_cvs = base_cvs.length() # MPointArray()
out_mat = np.ones([itGeo.count(), num_cvs])
bone_aim_axis = np.array([1,0,0,0]) # hardcoded for now : bones are oriented in +X
while not itGeo.isDone():
i = itGeo.index()
vtx_pos = itGeo.position()
for j in xrange(num_cvs):
base_cv = base_cvs[j]
offset_cv= base_cv + om.MVector(offsets[i])
base_mat = self.MMatrix_to_np_mat(base_mat_bones[j])
# get the normalized base vector cv->vertex
cv_to_pos = vtx_pos - offset_cv
np_cv_to_pos = np.array([cv_to_pos.x, cv_to_pos.y, cv_to_pos.z])
if np.linalg.norm(np_cv_to_pos) != 0:
np_cv_to_pos = np_cv_to_pos / np.linalg.norm(np_cv_to_pos)
# get the orientation (i.e. should we push the CV in x or pull it?)
base_bone_orient_pos = self.filter_matrix_axis(base_mat, bone_aim_axis)
base_bone_orient_pos = base_bone_orient_pos / np.linalg.norm(base_bone_orient_pos)
base_bone_orient_neg = -base_bone_orient_pos
pos_x = np_cv_to_pos.dot(base_bone_orient_pos)
neg_x = np_cv_to_pos.dot(base_bone_orient_neg)
if pos_x > neg_x:
out_mat[i, j] = +1
else:
out_mat[i, j] = -1
else:
out_mat[i, j] = 0
itGeo.next()
itGeo.reset()
return out_mat
def offset_CVs_by_tau(self, pos, offset_cvs, mat_bones, tau, cv_weights, cv_direction):
'''
We computed, in the init, whether we should pull or push the CV.
To know of how much we move the CV, we multiply the current bone aim
vector by the pre-computed direction (i.e. +1 or -1) by a remapped value of 'tau'
:param offset_cvs: offset cvs, after we applied the delta vector to them
'''
vtx_pos = np.array([pos.x, pos.y, pos.z])
bone_aim_axis = np.array([1,0,0,0]) # hardcoded for now : bones are oriented in +X
num_cvs = len(offset_cvs)
out_cvs = np.zeros([num_cvs, 3])
for i in xrange(num_cvs):
offset_cv = offset_cvs[i]
mat_bone = self.MMatrix_to_np_mat(mat_bones[i])
bone_aim_vector = self.filter_matrix_axis(mat_bone, bone_aim_axis)
bone_aim_vector = bone_aim_vector / np.linalg.norm(bone_aim_vector)
# offset_vector = om.MPoint(*(bone_aim_vector * cv_weights[i] * cv_direction[i] * tau))
offset_vector = om.MPoint(*(bone_aim_vector * cv_weights[i] * tau * -1))
new_cv = om.MPoint(*offset_cv) + om.MVector(offset_vector)
out_cvs[i] = [new_cv.x, new_cv.y, new_cv.z]
return out_cvs
# ---------------------- No longer used ------------------------
def weight_with_rbf(self, n, point, sigma=1):
num_points = len(n)
f = np.zeros([num_points])
for i in xrange(num_points):
pose = n[i]
dist = np.linalg.norm(pose-point)
gaussian = np.exp(-np.power(dist*sigma, 2))
f[i] = gaussian
ii=np.zeros([num_points, num_points])
for i in xrange(num_points):
jj=[]
posei=n[i]
for j in xrange(num_points):
posej=n[j]
dist = np.linalg.norm(posei-posej)
gaussian = np.exp(-np.power(dist, 2))
ii[j, i] = gaussian
poseWeights = np.linalg.solve(ii, f)
return poseWeights
def assign_weight_per_offset_cv(self, itGeo, base_cvs, deltas):
'''
Assigns a weight for each offset CV, based on the invert distance to
the deformed vertex
TODO : when the CV is on the vertex, we encounter a division by 0.
Need to fix that
:param itGeo: geo iterator
:type itGeo: MItGeometry
:param base_cvs: position of the CVs of the base curve
(i.e. list of n 3D-coord, with n=number of CVs)
:type base_cvs: MPointArray
:param deltas: delta b/w the deformed vertex and the closest pt on crv
:type deltas: MPointArray
'''
num_cvs = base_cvs.length()
weights_mat = np.zeros([itGeo.count(), num_cvs])
while not itGeo.isDone():
# first, offset the curve (and convert everything to numpy...)
offset_cvs = np.zeros([num_cvs])
delta = np.array([deltas[itGeo.index()].x,
deltas[itGeo.index()].y,
deltas[itGeo.index()].z])
vtx_pos = np.array([itGeo.position().x,
itGeo.position().y,
itGeo.position().z])
weight_sum = 0
for i in xrange(num_cvs):
cv = np.array([base_cvs[i].x, base_cvs[i].y, base_cvs[i].z])
# offset_cv = cv + delta
# then, weight it
inv_dist = 1./ np.power(np.linalg.norm(vtx_pos-cv), 4)
offset_cvs[i] = inv_dist
weight_sum += inv_dist
norm_offset_cvs = offset_cvs / weight_sum
# and finally, store the weights of this curve in a matrix of
# m x n where m=number of vertices and n=number of CVs of the base crv
weights_mat[itGeo.index()] = norm_offset_cvs
itGeo.next()
itGeo.reset()
return weights_mat
def assign_weight_per_cv(self, O, base_cvs):
num_cvs = base_cvs.length()
weights_vec = np.zeros([num_cvs])
weight_sum = 0.0
for i in xrange(num_cvs):
cv = np.array([base_cvs[i].x, base_cvs[i].y, base_cvs[i].z])
# then, weight it
dist = np.linalg.norm(O - cv)
inv_dist = 1./ np.power(dist, 4)
weights_vec[i] = inv_dist
weight_sum += inv_dist
weights_vec = weights_vec / weight_sum
return weights_vec
# ---------------------- debug/convenient functions ------------------------
def filter_matrix_axis(self, matrix, vector):
'''
Returns the correct axis of the matrix, based on the input vector.
Ex : on a matrix [[1,2,3,0], [4,5,6,0], [7,8,9,0], [0,0,0,1]], with the
vector [0,1,0,0], will return only the Y axis, [4,5,6]
:param matrix: input matrix we want to filter
:type matrix: np.array (in 2D)
:param vector: vector array used to filter the matrix
:type vector: np.array
'''
for axis in xrange(len(matrix)):
output = matrix[axis] * vector[axis]
if any([output[i] != 0 for i in xrange(4)]):
return output[:3]
def MMatrix_to_np_mat(self, matrix):
return np.array([[matrix(j, i) for i in xrange(4)] for j in xrange(4)])
def _remap(self, value, oldMin, oldMax, newMin, newMax):
return (((value - oldMin) * (newMax - newMin)) / (oldMax - oldMin)) + newMin
def printVector(self, v):
if isinstance(v, om.MPoint) or isinstance(v, om.MVector):
return [round(x, 2) for x in (v.x, v.y, v.z)]
else:
return [round(x, 2) for x in v]
def printMat(self, mat):
for i in xrange(4):
print [round(mat(i, j), 2) for j in xrange(4)]
def draw_point(self, point, color=[255, 255, 0]):
raise DeprecationWarning ('No longer useful, since we use VP2.0')
# view = OpenMayaUI.M3dView.active3dView()
# view.beginGL()
# glFT.glPointSize(5.8)
# glFT.glBegin(OpenMayaRender.MGL_POINTS)
# glFT.glColor3f(*color)
# if isinstance(point, om.MPoint) or isinstance(point, om.MVector):
# glFT.glVertex3f(point.x, point.y, point.z)
# else:
# glFT.glVertex3f(point[0], point[1], point[2])
# glFT.glEnd()
# offset = .01
# if isinstance(point, om.MPoint) or isinstance(point, om.MVector):
# glFT.glBegin(OpenMayaRender.MGL_LINES)
# glFT.glVertex3f(point.x-offset, point.y, point.z)
# glFT.glVertex3f(point.x+offset, point.y, point.z)
# glFT.glEnd()
# glFT.glBegin(OpenMayaRender.MGL_LINES)
# glFT.glVertex3f(point.x, point.y-offset, point.z)
# glFT.glVertex3f(point.x, point.y+offset, point.z)
# glFT.glEnd()
# glFT.glBegin(OpenMayaRender.MGL_LINES)
# glFT.glVertex3f(point.x-offset, point.y, point.z-offset)
# glFT.glVertex3f(point.x+offset, point.y, point.z+offset)
# glFT.glEnd()
# else:
# glFT.glBegin(OpenMayaRender.MGL_LINES)
# glFT.glVertex3f(point[0]-offset, point[1], point[2])
# glFT.glVertex3f(point[0]+offset, point[1], point[2])
# glFT.glEnd()
# glFT.glBegin(OpenMayaRender.MGL_LINES)
# glFT.glVertex3f(point[0], point[1]-offset, point[2])
# glFT.glVertex3f(point[0], point[1]+offset, point[2])
# glFT.glEnd()
# glFT.glBegin(OpenMayaRender.MGL_LINES)
# glFT.glVertex3f(point[0]-offset, point[1], point[2]-offset)
# glFT.glVertex3f(point[0]+offset, point[1], point[2]+offset)
# glFT.glEnd()
# view.endGL()
def draw_vector(self, v, pos=[0,0,0], color=[255,255,255]):
raise DeprecationWarning ('No longer useful, since we use VP2.0')
# view = OpenMayaUI.M3dView.active3dView()
# view.beginGL()
# glFT.glColor3f(*color)
# glFT.glBegin(OpenMayaRender.MGL_LINES)
# if isinstance(pos, om.MPoint) or isinstance(pos, om.MVector):
# start = [pos.x, pos.y, pos.z]
# else:
# start = pos
# if isinstance(v, om.MPoint) or isinstance(v, om.MVector):
# end = [start[0] + v.x, start[0] + v.y, start[0] + v.z]
# else:
# end = [start[0] + v[0], start[1] + v[1], start[2] + v[2]]
# glFT.glVertex3f(*start)
# glFT.glVertex3f(*end)
# glFT.glEnd()
# view.endGL()
def draw_curve(self, nurbsCurve, color=[randint(0,100)/100. for _ in xrange(3)]):
raise DeprecationWarning ('No longer useful, since we use VP2.0')
# view = OpenMayaUI.M3dView.active3dView()
# view.beginGL()
# pts = nurbsCurve.compute_crv()
# for i in xrange(len(pts)):
# if i == 0:
# continue
# glFT.glColor3f(*color)
# glFT.glLineWidth(1)
# glFT.glBegin(OpenMayaRender.MGL_LINES)
# glFT.glVertex3f(pts[i-1][0], pts[i-1][1], pts[i-1][2])
# glFT.glVertex3f(pts[i][0], pts[i][1], pts[i][2])
# glFT.glEnd()
# view.endGL()
def nodeCreator():
return omMpx.asMPxPtr(curveDeformer())
def nodeInitializer():
tAttr = om.MFnTypedAttribute()
nAttr = om.MFnNumericAttribute()
mAttr = om.MFnMatrixAttribute()
cAttr = om.MFnCompoundAttribute()
# init
curveDeformer.aInit = nAttr.create('initialize', 'init', om.MFnNumericData.kBoolean, True)
curveDeformer.addAttribute(curveDeformer.aInit)
nAttr.setChannelBox(True)
# inCurve
curveDeformer.aInCrv = tAttr.create('inputCurve', 'inCrv', om.MFnData.kNurbsCurve)
curveDeformer.addAttribute(curveDeformer.aInCrv)
# baseCurve
curveDeformer.aBaseCrv = tAttr.create('baseCurve', 'baseCrv', om.MFnData.kNurbsCurve)
curveDeformer.addAttribute(curveDeformer.aBaseCrv)
# curve controllers - now used only for tweaking the weight of each CV
curveDeformer.aWeight = nAttr.create('weight', 'wgt', om.MFnNumericData.kFloat, 1.)
nAttr.setKeyable(True)
nAttr.setMin(.001)
curveDeformer.aCps = cAttr.create('controlPoints', 'cps')
cAttr.addChild(curveDeformer.aWeight)
cAttr.setArray(True)
curveDeformer.addAttribute(curveDeformer.aCps)
# connected joints (used to compute Tau)
curveDeformer.aMatrixJoint = mAttr.create('matrixJoint', 'matJt')
curveDeformer.aMatrixJoints = cAttr.create('matrixJoints', 'matJts')
cAttr.addChild(curveDeformer.aMatrixJoint)
cAttr.setArray(True)
curveDeformer.addAttribute(curveDeformer.aMatrixJoints)
# attribute effects
curveDeformer.attributeAffects(curveDeformer.aInit, curveDeformer.outputGeom)
curveDeformer.attributeAffects(curveDeformer.aInCrv, curveDeformer.outputGeom)
curveDeformer.attributeAffects(curveDeformer.aBaseCrv, curveDeformer.outputGeom)
curveDeformer.attributeAffects(curveDeformer.aCps, curveDeformer.outputGeom)
curveDeformer.attributeAffects(curveDeformer.aMatrixJoints, curveDeformer.outputGeom)
# make deformer paintable
om.MGlobal.executeCommand("makePaintable -attrType multiFloat -sm deformer curveDeformer ws;")
def initializePlugin(mObj):
plugin = omMpx.MFnPlugin(mObj, 'fruity', '1.0', 'any')
try:
plugin.registerNode(pluginName, pluginId, nodeCreator, nodeInitializer, omMpx.MPxNode.kDeformerNode)
except:
sys.stderr.write('Load plugin failed: %s' % pluginName)
def uninitializePlugin(mObj):
plugin = omMpx.MFnPlugin(mObj)
try:
plugin.deregisterNode(pluginId)
except:
sys.stderr.write('Unload plugin failed: %s' % pluginName)
<file_sep>/src/nurbsCurve.py
import numpy as np
class NurbsCurve(object):
'''
https://fr.wikipedia.org/wiki/NURBS#Les_courbes_NURBS
https://en.wikipedia.org/wiki/De_Boor%27s_algorithm#Local_support
a = NurbsCurve(points=([10,10,0], [5,10,2], [-5,5,0], [10,5,-2], [4,10,0], [4,5,2], [8,1,0]), knots=[0,0,0,0,1,2,3,4,4,4,4], degree=3)
a.draw_crv()
param = .5
pt_at_param = a.pt_at_param(param)
tan_at_param = a.tan_at_param(param)
a.compute_crv()
a.draw_crv()
'''
def __init__(self, points, knots, degree, weights=None, LOD=20):
'''
:param points: array of position of each 3d point (Control Point)
:type points: array(float3)
:param knots: knot vector (of length number of CVs + degree + 1)
:type knots: array(float)
:param degree: degree of the curve
:type degree: int
:param weights: array of int representing the weights of each CV
:type weights: array(int)
:param LOD: Level of detail - if we compute the curve, we'll compute
LOD points on the curve. With LOD=20, our full curve
will have 20 out points. Useful only if we want to draw
the curve
:type LOD: int
'''
self._cvs = np.asarray(points)
self._num_cvs = len(self._cvs)
self._degree = degree
self._order = self._degree + 1
self._num_knots = self._num_cvs + self._degree + 1
self._LOD = LOD
self._knots = knots
self._out_pts = None # the curve hasn't been computed yet
self._weights = weights or np.ones(self._num_cvs)
def compute_crv(self):
'''
Computes the curve at n parameters (with n=LOD). Running this will
populate _out_pts, that we can use to draw the curve if needed
'''
self._out_pts = []
for i in xrange(self._LOD):
t = self._knots[self._num_knots-1] * i / (self._LOD-1.)
if i == self._LOD-1:
t -= .0001
out_point = self.pt_at_param(t)
# out_tangent = self.tan_at_param(t)
self._out_pts.append(out_point)
return self._out_pts
def _CoxDeBoor(self, t, i, k, knots):
'''
Recursive function to find the value N affecting the current parameter
:param t: parameter
:type t: float
:param i: index of the CV we treat currently
:type i: int
:param k: order (i.e. degree + 1 by default, on which we removes 1
until k = 1). The order is the parameter to change to have
an open / close / periodic curve
:type k: int
:param knots: knot vector.
:type knots: list of int
:return:
'''
if k == 1:
if knots[i] <= t and t <= knots[i+1]:
return 1.
else:
return 0.
denominator1 = knots[i+k-1] - knots[i]
denominator2 = knots[i+k] - knots[i+1]
Eq1 = 0
Eq2 = 0
if denominator1 > 0:
Eq1 = ((t-knots[i]) / denominator1) * self._CoxDeBoor(t, i, k-1, knots)
if denominator2 > 0:
Eq2 = (knots[i+k]-t) / denominator2 * self._CoxDeBoor(t, i+1, k-1, knots)
return Eq1 + Eq2
def pt_at_param(self, t):
'''
Returns the float3 position of a point at the given parameter t
:param t: parameter we query
:type t: float
:return : position of the point in 3D
:return type: np.array()
'''
# sum of the effect of all the CVs on the curve at the given parameter
# to get the evaluated curve point
numerator = np.zeros(3)
denominator = 0
for i in xrange(self._num_cvs):
# compute the effect of this point on the curve
N = self._CoxDeBoor(t, i, self._order, self._knots)
if N > .0001:
numerator += (self._weights[i] * self._cvs[i] * N)
denominator += (self._weights[i] * N)
return numerator / denominator
'''
# If we don't need to have weighted values, we can use this equation
# to make thigns faster
for i in xrange(self._num_cvs):
val = self._CoxDeBoor(t, i, self._order, self._knots)
if val > .001:
# sum effect of CV on this part of the curve
out_pt[0] += val * self._cvs[i][0]
out_pt[1] += val * self._cvs[i][1]
out_pt[2] += val * self._cvs[i][2]
'''
def tan_at_param(self, t):
'''
Returns the vector of the tangent at the given parameter t
:param t: parameter we query
:type t: float
:return : position of the point in 3D
:return type: np.array()
'''
numerator1A = np.zeros(3)
numerator1B = np.zeros(3)
numerator2A = np.zeros(3)
numerator2B = np.zeros(3)
denominator = 0
for i in xrange(self._num_cvs):
N = self._CoxDeBoor(t, i, self._order, self._knots)
dN = self._CoxDeBoorDerived(t, i, self._order, self._knots)
if N > .0001:
denominator += (self._weights[i] * N)
# first equation
numerator1A += (self._weights[i] * self._cvs[i] * dN)
numerator1B += (self._weights[i] * N)
# second equation
numerator2A += (self._weights[i] * self._cvs[i] * N)
numerator2B += (self._weights[i] * dN)
eq1 = (numerator1A * numerator1B) / (denominator**2)
eq2 = (numerator2A * numerator2B) / (denominator**2)
return eq1 - eq2
def _CoxDeBoorDerived(self, t, i, k, knots):
'''
Derivated function of the _CoxDeBoor algorithm, used only for the tangent
:param t: parameter
:type t: float
:param i: index of the CV we treat currently
:type i: int
:param k: order (i.e. degree + 1 by default, on which we removes 1
until k = 1). The order is the parameter to change to have
an open / close / periodic curve
:type k: int
:param knots: knot vector.
:type knots: list of int
:return:
'''
if k == 1:
if knots[i] <= t and t <= knots[i+1]:
return 1.
else:
return 0.
denominator1 = knots[i+k-1] - knots[i]
denominator2 = knots[i+k] - knots[i+1]
Eq1 = 0
Eq2 = 0
if denominator1 > 0:
Eq1 = (k / denominator1) * self._CoxDeBoor(t, i, k-1, knots)
if denominator2 > 0:
Eq2 = (k / denominator2) * self._CoxDeBoor(t, i+1, k-1, knots)
return Eq1 - Eq2
def draw_crv(self):
'''
Convenient function to compare our result with the same parameters
using maya curve
'''
cmds.curve(n='mayaCrv', d=self._degree, p=self._cvs, k=self._knots[1:-1])
cmds.curve(n='myCrv', d=1, p=self._out_pts)
| ba04002d6e6d8ed5ccaaafb624b26ed77659ba56 | [
"Python"
] | 2 | Python | moChen0607/curveDeformer | 88e090a3ac449310fd5651c0b8fd8e1499a513a9 | 5b17ccb397f27a40256bb345f8a775f07be4660e |
refs/heads/main | <repo_name>Ghazali-telecom/copy_past<file_sep>/requirements.txt
torchvision>=0.3.0
pretrainedmodels==0.7.4
efficientnet-pytorch==0.6.3
timm==0.3.2
<file_sep>/README.md
# Copy-Paste
Unofficial implementation of the copy-paste augmentation from [Simple Copy-Paste is a Strong Data Augmentation Method for Instance Segmentation](https://arxiv.org/abs/2012.07177v1).
The augmentation function is built to integrate easily with albumentations. An example for creating a compatible torchvision dataset is given for COCO. Core functionality for image, masks, and bounding boxes is finished; keypoints are not yet supported. In general, you can use the CopyPaste augmentation just as you would any other albumentations augmentation function. There are a few usage limitations of note.
<figure>
<img src="./example.png"></img>
</figure>
## Usage Notes
1. BboxParams cannot have label_fields. To attach class labels to a bounding box, directly append it to the bounding box coordinates. (I.e. (x1, y1, x2, y2, class_id)).
2. Bounding boxes passed to the CopyPaste augmentation must also include the index of the corresponding mask in the 'masks' list. (I.e. the bounding box looks like (x1, y1, x2, y2, class_id, mask_index)). An example is given for COCO.
3. The CopyPaste augmentation expects 6 keyword arguments instead of three:
```python
output = transforms(image=image, masks=masks, bboxes=bboxes)
--->instead
output = transforms(
image=image, masks=masks, bboxes=bboxes,
paste_image=paste_image, paste_masks=paste_masks, paste_bboxes=paste_bboxes
)
```
4. After pasting objects, the original bounding boxes may be occluded. To make things easier,
the original bounding boxes are just extracted from the updated masks.
## Integration with Torchvision datasets
The idea is to have a standard torchvision dataset that's decorated to add seamlessly integrate the
copy-paste functionality.
The dataset class looks like:
```python
from copy_paste import copy_paste_class
from torch.utils.data import Dataset
@copy_paste_class
class SomeVisionDataset(Dataset):
def __init__(self, *args):
super(SomeVisionDataset, self).__init__(*args)
def __len__(self):
return length
def load_example(self, idx):
image_data_dict = load_some_data(idx)
transformed_data_dict = self.transforms(**image_data_dict)
return transformed_data_dict
```
The only difference from a regular torchvision dataset is the decorator and the ```load_example``` method
instead of ```__getitem__```.
To compose transforms with copy-paste augmentation (bbox params are NOT optional):
```python
import albumentations as A
from albumentations.pytorch.transforms import ToTensorV2
from copy_paste import CopyPaste
transform = A.Compose([
A.RandomScale(scale_limit=(-0.9, 1), p=1), #LargeScaleJitter from scale of 0.1 to 2
A.PadIfNeeded(256, 256, border_mode=0), #constant 0 border
A.RandomCrop(256, 256),
A.HorizontalFlip(p=0.5),
CopyPaste(blend=True, sigma=1, pct_objects_paste=0.5, p=1)
], bbox_params=A.BboxParams(format="coco")
)
```
<file_sep>/copy_past/copy_paste.py
import os
import cv2
import random
import numpy as np
import albumentations as A
from copy import deepcopy
from skimage.filters import gaussian
def image_copy_paste(img, paste_img, alpha, blend=True, sigma=1):
if alpha is not None:
if blend:
alpha = gaussian(alpha, sigma=sigma, preserve_range=True)
img_dtype = img.dtype
alpha = alpha[..., None]
img = paste_img * alpha + img * (1 - alpha)
img = img.astype(img_dtype)
return img
def mask_copy_paste(mask, paste_mask, alpha):
raise NotImplementedError
def masks_copy_paste(masks, paste_masks, alpha):
if alpha is not None:
#eliminate pixels that will be pasted over
masks = [
np.logical_and(mask, np.logical_xor(mask, alpha)).astype(np.uint8) for mask in masks
]
masks.extend(paste_masks)
return masks
def extract_bboxes(masks):
bboxes = []
h, w = masks[0].shape
for mask in masks:
yindices = np.where(np.any(mask, axis=0))[0]
xindices = np.where(np.any(mask, axis=1))[0]
if yindices.shape[0]:
y1, y2 = yindices[[0, -1]]
x1, x2 = xindices[[0, -1]]
y2 += 1
x2 += 1
y1 /= w
y2 /= w
x1 /= h
x2 /= h
else:
y1, x1, y2, x2 = 0, 0, 0, 0
bboxes.append((y1, x1, y2, x2))
return bboxes
def bboxes_copy_paste(bboxes, paste_bboxes, masks, paste_masks, alpha, key):
if key == 'paste_bboxes':
return bboxes
elif paste_bboxes is not None:
masks = masks_copy_paste(masks, paste_masks=[], alpha=alpha)
adjusted_bboxes = extract_bboxes(masks)
#only keep the bounding boxes for objects listed in bboxes
mask_indices = [box[-1] for box in bboxes]
adjusted_bboxes = [adjusted_bboxes[idx] for idx in mask_indices]
#append bbox tails (classes, etc.)
adjusted_bboxes = [bbox + tail[4:] for bbox, tail in zip(adjusted_bboxes, bboxes)]
#adjust paste_bboxes mask indices to avoid overlap
if len(masks) > 0:
max_mask_index = len(masks)
else:
max_mask_index = 0
paste_mask_indices = [max_mask_index + ix for ix in range(len(paste_bboxes))]
paste_bboxes = [pbox[:-1] + (pmi,) for pbox, pmi in zip(paste_bboxes, paste_mask_indices)]
adjusted_paste_bboxes = extract_bboxes(paste_masks)
adjusted_paste_bboxes = [apbox + tail[4:] for apbox, tail in zip(adjusted_paste_bboxes, paste_bboxes)]
bboxes = adjusted_bboxes + adjusted_paste_bboxes
return bboxes
def keypoints_copy_paste(keypoints, paste_keypoints, alpha):
#remove occluded keypoints
if alpha is not None:
visible_keypoints = []
for kp in keypoints:
x, y = kp[:2]
tail = kp[2:]
if alpha[int(y), int(x)] == 0:
visible_keypoints.append(kp)
keypoints = visible_keypoints + paste_keypoints
return keypoints
class CopyPaste(A.DualTransform):
def __init__(
self,
blend=True,
sigma=3,
pct_objects_paste=0.1,
max_paste_objects=None,
p=0.5,
always_apply=False
):
super(CopyPaste, self).__init__(always_apply, p)
self.blend = blend
self.sigma = sigma
self.pct_objects_paste = pct_objects_paste
self.max_paste_objects = max_paste_objects
self.p = p
self.always_apply = always_apply
@staticmethod
def get_class_fullname():
return 'copypaste.CopyPaste'
@property
def targets_as_params(self):
return [
"masks",
"paste_image",
#"paste_mask",
"paste_masks",
"paste_bboxes",
#"paste_keypoints"
]
def get_params_dependent_on_targets(self, params):
image = params["paste_image"]
masks = None
if "paste_mask" in params:
#handle a single segmentation mask with
#multiple targets
#nothing for now.
raise NotImplementedError
elif "paste_masks" in params:
masks = params["paste_masks"]
assert(masks is not None), "Masks cannot be None!"
bboxes = params.get("paste_bboxes", None)
keypoints = params.get("paste_keypoints", None)
#number of objects: n_bboxes <= n_masks because of automatic removal
n_objects = len(bboxes) if bboxes is not None else len(masks)
#paste all objects if no restrictions
n_select = n_objects
if self.pct_objects_paste:
n_select = int(n_select * self.pct_objects_paste)
if self.max_paste_objects:
n_select = min(n_select, self.max_paste_objects)
#no objects condition
if n_select == 0:
return {
"param_masks": params["masks"],
"paste_img": None,
"alpha": None,
"paste_mask": None,
"paste_masks": None,
"paste_bboxes": None,
"paste_keypoints": None,
"objs_to_paste": []
}
#select objects
objs_to_paste = np.random.choice(
range(0, n_objects), size=n_select, replace=False
)
#take the bboxes
if bboxes:
bboxes = [bboxes[idx] for idx in objs_to_paste]
#the last label in bboxes is the index of corresponding mask
mask_indices = [bbox[-1] for bbox in bboxes]
#create alpha by combining all the objects into
#a single binary mask
masks = [masks[idx] for idx in mask_indices]
alpha = masks[0] > 0
for mask in masks[1:]:
alpha += mask > 0
return {
"param_masks": params["masks"],
"paste_img": image,
"alpha": alpha,
"paste_mask": None,
"paste_masks": masks,
"paste_bboxes": bboxes,
"paste_keypoints": keypoints
}
@property
def ignore_kwargs(self):
return [
"paste_image",
"paste_mask",
"paste_masks"
]
def apply_with_params(self, params, force_apply=False, **kwargs): # skipcq: PYL-W0613
if params is None:
return kwargs
params = self.update_params(params, **kwargs)
res = {}
for key, arg in kwargs.items():
if arg is not None and key not in self.ignore_kwargs:
target_function = self._get_target_function(key)
target_dependencies = {k: kwargs[k] for k in self.target_dependence.get(key, [])}
target_dependencies['key'] = key
res[key] = target_function(arg, **dict(params, **target_dependencies))
else:
res[key] = None
return res
def apply(self, img, paste_img, alpha, **params):
return image_copy_paste(
img, paste_img, alpha, blend=self.blend, sigma=self.sigma
)
def apply_to_mask(self, mask, paste_mask, alpha, **params):
return mask_copy_paste(mask, paste_mask, alpha)
def apply_to_masks(self, masks, paste_masks, alpha, **params):
return masks_copy_paste(masks, paste_masks, alpha)
def apply_to_bboxes(self, bboxes, paste_bboxes, param_masks, paste_masks, alpha, key, **params):
return bboxes_copy_paste(bboxes, paste_bboxes, param_masks, paste_masks, alpha, key)
def apply_to_keypoints(self, keypoints, paste_keypoints, alpha, **params):
raise NotImplementedError
#return keypoints_copy_paste(keypoints, paste_keypoints, alpha)
def get_transform_init_args_names(self):
return (
"blend",
"sigma",
"pct_objects_paste",
"max_paste_objects"
)
def copy_paste_class(dataset_class):
def _split_transforms(self):
split_index = None
for ix, tf in enumerate(list(self.transforms.transforms)):
if tf.get_class_fullname() == 'copypaste.CopyPaste':
split_index = ix
if split_index is not None:
tfs = list(self.transforms.transforms)
pre_copy = tfs[:split_index]
copy_paste = tfs[split_index]
post_copy = tfs[split_index+1:]
#replicate the other augmentation parameters
bbox_params = None
keypoint_params = None
paste_additional_targets = {}
if 'bboxes' in self.transforms.processors:
bbox_params = self.transforms.processors['bboxes'].params
paste_additional_targets['paste_bboxes'] = 'bboxes'
if self.transforms.processors['bboxes'].params.label_fields:
msg = "Copy-paste does not support bbox label_fields! "
msg += "Expected bbox format is (a, b, c, d, label_field)"
raise Exception(msg)
if 'keypoints' in self.transforms.processors:
keypoint_params = self.transforms.processors['keypoints'].params
paste_additional_targets['paste_keypoints'] = 'keypoints'
if keypoint_params.label_fields:
raise Exception('Copy-paste does not support keypoint label fields!')
if self.transforms.additional_targets:
raise Exception('Copy-paste does not support additional_targets!')
#recreate transforms
self.transforms = A.Compose(pre_copy, bbox_params, keypoint_params, additional_targets=None)
self.post_transforms = A.Compose(post_copy, bbox_params, keypoint_params, additional_targets=None)
self.copy_paste = A.Compose(
[copy_paste], bbox_params, keypoint_params, additional_targets=paste_additional_targets
)
else:
self.copy_paste = None
self.post_transforms = None
def __getitem__(self, idx):
#split transforms if it hasn't been done already
if not hasattr(self, 'post_transforms'):
self._split_transforms()
img_data = self.load_example(idx)
if self.copy_paste is not None:
paste_idx = random.randint(0, self.__len__() - 1)
paste_img_data = self.load_example(paste_idx)
for k in list(paste_img_data.keys()):
paste_img_data['paste_' + k] = paste_img_data[k]
del paste_img_data[k]
img_data = self.copy_paste(**img_data, **paste_img_data)
img_data = self.post_transforms(**img_data)
img_data['paste_index'] = paste_idx
return img_data
setattr(dataset_class, '_split_transforms', _split_transforms)
setattr(dataset_class, '__getitem__', __getitem__)
return dataset_class
if __name__ == '__main__':
print('Hello') | 77e44ef33245725b8b5d8d913e0e761045c8cc63 | [
"Markdown",
"Python",
"Text"
] | 3 | Text | Ghazali-telecom/copy_past | 290c1b440c6a42b50dd55e4cc3ff2c7038ddd61f | bc995af970255e374b9a124e477beb7a63c55711 |
refs/heads/master | <repo_name>sanjump/RelianceDigital_Scraping<file_sep>/RelianceDigital_Electronics/RelianceDigital_Electronics/spiders/camera.py
import scrapy,random,string
from ..items import ReliancedigitalElectronicsItem
class RelianceSpider(scrapy.Spider):
name = 'reliancecamera'
pageno=2
start_urls=['https://www.reliancedigital.in/dslr-cameras/c/S101110?searchQuery=:relevance:availability:Exclude%20out%20of%20Stock&page=0']
def parse(self, response, **kwargs):
page = response.css(".sp a::attr('href')").getall()
for p in page:
url = 'https://www.reliancedigital.in' + p
yield scrapy.Request(url, callback=self.parse_elec)
for page in ['https://www.reliancedigital.in/mirrorlesss-cameras/c/S101119?searchQuery=:relevance:availability:Exclude%20out%20of%20Stock&page=0',
'https://www.reliancedigital.in/point-shoot-cameras/c/S101111?searchQuery=:relevance:availability:Exclude%20out%20of%20Stock&page=0',
'https://www.reliancedigital.in/prosumer-cameras/c/S101112?searchQuery=:relevance:availability:Exclude%20out%20of%20Stock&page=0',
'https://www.reliancedigital.in/action-cameras/c/S101113?searchQuery=:relevance:availability:Exclude%20out%20of%20Stock&page=0']:
yield response.follow(page, callback=self.parse)
def parse_elec(self, response):
items = ReliancedigitalElectronicsItem()
product_name = response.css('.pdp__title::text').get()
storeprice = response.css('.pdp__offerPrice::text').extract()
storeLink = response.url
photos = response.css(".pdp__mainHeroImgContainer::attr('data-srcset')").get()
id = storeLink[::-1][:storeLink[::-1].find('/')]
#rating = response.css('.hGSR34::text').extract()
#reviews = response.css('.qwjRop div div::text').extract()
product_id = ''.join(random.sample(string.ascii_lowercase + string.digits, 20))
spec_title = response.css(".pdp__tab-info__list__name::text").extract()
spec_detail = response.css(".pdp__tab-info__list__value::text").extract()
stores = {
"rating" : "NA",
"reviews" : [],
"storeProductId": id[::-1],
"storeLink": storeLink,
"storeName": "RelianceDigital",
"storePrice": storeprice[0][1:]
}
items['product_name'] = product_name
items['product_id'] = product_id
items['stores'] = stores
items['category'] = 'electronics'
items['subcategory'] = 'cameras'
items['brand'] = product_name.split()[0]
items['description']={}
for i in range(len(spec_title)):
items['description'][spec_title[i]] = spec_detail[i]
items["photos"] = 'https://www.reliancedigital.in'+ photos
yield items | 879e50080e42a61e0c75def179371548bd44e40c | [
"Python"
] | 1 | Python | sanjump/RelianceDigital_Scraping | 044d099de8d322998ee4a700d1e4e4b045a88ebb | a9935d0c2887147092ac0fa3edd8c54ce1363d79 |
refs/heads/master | <repo_name>OctoConsulting/EventSourceExample<file_sep>/src/main/java/com/octo/EventSourceExample/command/RequestCommandServiceImpl.java
package com.octo.EventSourceExample.command;
import java.util.UUID;
import java.util.concurrent.CompletableFuture;
import org.axonframework.commandhandling.gateway.CommandGateway;
import org.springframework.stereotype.Service;
@Service
public class RequestCommandServiceImpl implements RequestCommandService {
private final CommandGateway commandGateway;
public RequestCommandServiceImpl(CommandGateway commandGateway) {
this.commandGateway = commandGateway;
}
@Override
public CompletableFuture<String> createRequest(RequestCreateDTO requestCreateDTO) {
return commandGateway.send(new CreateRequestCommand(UUID.randomUUID().toString(), requestCreateDTO.getCreatedBy(),
requestCreateDTO.getCategory()));
}
@Override
public CompletableFuture<String> deleteRequest(RequestDeleteDTO requestDeleteDTO) {
return commandGateway.send(new DeleteRequestCommand(requestDeleteDTO.getId()));
}
}
<file_sep>/src/main/java/com/octo/EventSourceExample/query/RequestQueryServiceImpl.java
package com.octo.EventSourceExample.query;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
import org.axonframework.eventsourcing.eventstore.EventStore;
import org.springframework.stereotype.Service;
@Service
public class RequestQueryServiceImpl implements RequestQueryService {
private final EventStore eventStore;
private final RequestRepository requestRepository;
public RequestQueryServiceImpl(EventStore eventStore, RequestRepository requestRepository) {
this.eventStore = eventStore;
this.requestRepository = requestRepository;
}
@Override
public List<Object> listEventsForRequest(String requestId) {
return eventStore.readEvents(requestId).asStream().map(s -> s.getPayload()).collect(Collectors.toList());
}
@Override
public RequestQueryEntity getRequest(String requestId) {
return requestRepository.findById(requestId).get();
}
@Override
public List<RequestQueryEntity> getAllRequests() {
List<RequestQueryEntity> result = new ArrayList<RequestQueryEntity>();
requestRepository.findAll().forEach(r -> {
if (!r.isDeleted())
result.add(r);
});
return result;
}
}
<file_sep>/src/main/java/com/octo/EventSourceExample/query/RequestQueryService.java
package com.octo.EventSourceExample.query;
import java.util.List;
public interface RequestQueryService {
public List<Object> listEventsForRequest(String requestId);
public RequestQueryEntity getRequest(String requestId);
public List<RequestQueryEntity> getAllRequests();
}
<file_sep>/src/test/java/com/octo/EventSourceExample/EventSourceExampleApplicationTests.java
package com.octo.EventSourceExample;
import org.junit.jupiter.api.Test;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
class EventSourceExampleApplicationTests {
@Test
void contextLoads() {
}
}
<file_sep>/README.md
# EventSourceExample
This is an example repository for event sourcing using Axon and Spring Boot
<file_sep>/src/main/java/com/octo/EventSourceExample/query/RequestQueryController.java
package com.octo.EventSourceExample.query;
import java.util.List;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import io.swagger.annotations.Api;
@RestController
@RequestMapping(value = "/request")
@Api(value = "Request Queries", description = "Request Query Events Endpoint", tags = "Request Queries")
public class RequestQueryController {
private final RequestQueryService requestQueryService;
public RequestQueryController(RequestQueryService requestQueryService) {
this.requestQueryService = requestQueryService;
}
@GetMapping("/{requestId}")
public RequestQueryEntity getReqeust(@PathVariable(value = "requestId") String requestId) {
return requestQueryService.getRequest(requestId);
}
@GetMapping("/{requestId}/events")
public List<Object> listEventsForRequest(@PathVariable(value = "requestId") String requestId) {
return requestQueryService.listEventsForRequest(requestId);
}
@GetMapping("/all")
public List<RequestQueryEntity> getAllRequests() {
return requestQueryService.getAllRequests();
}
}
<file_sep>/src/main/java/com/octo/EventSourceExample/command/RequestCreateDTO.java
package com.octo.EventSourceExample.command;
public class RequestCreateDTO {
private String createdBy;
private String category;
public String getCreatedBy() {
return createdBy;
}
public String getCategory() {
return category;
}
public void setCategory(String category) {
this.category = category;
}
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
}
| 15daf55d9bf562fe5da03851b31ffb8314cb3d53 | [
"Markdown",
"Java"
] | 7 | Java | OctoConsulting/EventSourceExample | c42fb6a094f0663c7fb31cd5e32a0f2b8e91420f | 497c63ab062638f2ad3d6f288323318d7c479f53 |
refs/heads/master | <repo_name>ChinaMCat/grpcserver<file_sep>/AppServer.go
package main
import (
"net"
"fmt"
"google.golang.org/grpc"
"golang.org/x/net/context"
"grpctest/testg"
"io"
)
func main() {
listen,err := net.Listen("tcp",":50052")
if err != nil {
fmt.Println(err)
}
s := grpc.NewServer()
testg.RegisterUserServerServer(s,&UserServer{})
fmt.Println("启动")
s.Serve(listen)
}
func testListFunc() *testg.UserList {
uList := new(testg.UserList)
u := new(testg.User)
u.UserId = "1"
u.UserName = "小王"
u.Age = 20
uList.U = append(uList.U,u)
u1 := new(testg.User)
u1.UserId = "2"
u1.UserName = "小张"
u1.Age = 15
uList.U = append(uList.U,u1)
//fmt.Println(uList)
return uList
}
type UserServer struct {
}
func (u *UserServer) GetUserById(c context.Context,user *testg.User) (*testg.User, error) {
userId := user.UserId
if userId == "" {
return nil, nil
}
fmt.Println("userId:", userId)
ul := testListFunc()
for _,v := range ul.U{
if v.UserId == user.UserId {
return v, nil
}
}
return nil, nil
}
func (u *UserServer) GetList(c context.Context,e *testg.Empty) (*testg.UserList, error) {
return testListFunc(),nil
}
func (u *UserServer) GetListStream(e *testg.Empty, us testg.UserServer_GetListStreamServer) error {
ul := testListFunc()
for _,v := range ul.U{
us.Send(v)
}
return nil
}
func (u *UserServer) SetUserStream(us testg.UserServer_SetUserStreamServer) error {
for {
user,err := us.Recv()
if err == io.EOF {
return us.SendAndClose(testListFunc())
}
if err != nil {
return err
}
fmt.Println("user", user)
fmt.Println(user.UserName)
}
return nil
}
func (u *UserServer) Chat(us testg.UserServer_ChatServer) error {
for {
user,err := us.Recv()
if err == io.EOF {
return nil
}
fmt.Println("user",user)
us.Send(user)
}
return nil
}<file_sep>/testg/UserServerGrpc.java
package testg;
import static io.grpc.MethodDescriptor.generateFullMethodName;
import static io.grpc.stub.ClientCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ClientCalls.asyncClientStreamingCall;
import static io.grpc.stub.ClientCalls.asyncServerStreamingCall;
import static io.grpc.stub.ClientCalls.asyncUnaryCall;
import static io.grpc.stub.ClientCalls.blockingServerStreamingCall;
import static io.grpc.stub.ClientCalls.blockingUnaryCall;
import static io.grpc.stub.ClientCalls.futureUnaryCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.9.1)",
comments = "Source: testg/user_server.proto")
public final class UserServerGrpc {
private UserServerGrpc() {}
public static final String SERVICE_NAME = "testg.UserServer";
// Static method descriptors that strictly reflect the proto.
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
@java.lang.Deprecated // Use {@link #getGetUserByIdMethod()} instead.
public static final io.grpc.MethodDescriptor<testg.UserVo.User,
testg.UserVo.User> METHOD_GET_USER_BY_ID = getGetUserByIdMethod();
private static volatile io.grpc.MethodDescriptor<testg.UserVo.User,
testg.UserVo.User> getGetUserByIdMethod;
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static io.grpc.MethodDescriptor<testg.UserVo.User,
testg.UserVo.User> getGetUserByIdMethod() {
io.grpc.MethodDescriptor<testg.UserVo.User, testg.UserVo.User> getGetUserByIdMethod;
if ((getGetUserByIdMethod = UserServerGrpc.getGetUserByIdMethod) == null) {
synchronized (UserServerGrpc.class) {
if ((getGetUserByIdMethod = UserServerGrpc.getGetUserByIdMethod) == null) {
UserServerGrpc.getGetUserByIdMethod = getGetUserByIdMethod =
io.grpc.MethodDescriptor.<testg.UserVo.User, testg.UserVo.User>newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"testg.UserServer", "GetUserById"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
testg.UserVo.User.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
testg.UserVo.User.getDefaultInstance()))
.setSchemaDescriptor(new UserServerMethodDescriptorSupplier("GetUserById"))
.build();
}
}
}
return getGetUserByIdMethod;
}
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
@java.lang.Deprecated // Use {@link #getGetListMethod()} instead.
public static final io.grpc.MethodDescriptor<testg.UserVo.Empty,
testg.UserVo.UserList> METHOD_GET_LIST = getGetListMethod();
private static volatile io.grpc.MethodDescriptor<testg.UserVo.Empty,
testg.UserVo.UserList> getGetListMethod;
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static io.grpc.MethodDescriptor<testg.UserVo.Empty,
testg.UserVo.UserList> getGetListMethod() {
io.grpc.MethodDescriptor<testg.UserVo.Empty, testg.UserVo.UserList> getGetListMethod;
if ((getGetListMethod = UserServerGrpc.getGetListMethod) == null) {
synchronized (UserServerGrpc.class) {
if ((getGetListMethod = UserServerGrpc.getGetListMethod) == null) {
UserServerGrpc.getGetListMethod = getGetListMethod =
io.grpc.MethodDescriptor.<testg.UserVo.Empty, testg.UserVo.UserList>newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(
"testg.UserServer", "GetList"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
testg.UserVo.Empty.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
testg.UserVo.UserList.getDefaultInstance()))
.setSchemaDescriptor(new UserServerMethodDescriptorSupplier("GetList"))
.build();
}
}
}
return getGetListMethod;
}
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
@java.lang.Deprecated // Use {@link #getGetListStreamMethod()} instead.
public static final io.grpc.MethodDescriptor<testg.UserVo.Empty,
testg.UserVo.User> METHOD_GET_LIST_STREAM = getGetListStreamMethod();
private static volatile io.grpc.MethodDescriptor<testg.UserVo.Empty,
testg.UserVo.User> getGetListStreamMethod;
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static io.grpc.MethodDescriptor<testg.UserVo.Empty,
testg.UserVo.User> getGetListStreamMethod() {
io.grpc.MethodDescriptor<testg.UserVo.Empty, testg.UserVo.User> getGetListStreamMethod;
if ((getGetListStreamMethod = UserServerGrpc.getGetListStreamMethod) == null) {
synchronized (UserServerGrpc.class) {
if ((getGetListStreamMethod = UserServerGrpc.getGetListStreamMethod) == null) {
UserServerGrpc.getGetListStreamMethod = getGetListStreamMethod =
io.grpc.MethodDescriptor.<testg.UserVo.Empty, testg.UserVo.User>newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(
"testg.UserServer", "GetListStream"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
testg.UserVo.Empty.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
testg.UserVo.User.getDefaultInstance()))
.setSchemaDescriptor(new UserServerMethodDescriptorSupplier("GetListStream"))
.build();
}
}
}
return getGetListStreamMethod;
}
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
@java.lang.Deprecated // Use {@link #getSetUserStreamMethod()} instead.
public static final io.grpc.MethodDescriptor<testg.UserVo.User,
testg.UserVo.UserList> METHOD_SET_USER_STREAM = getSetUserStreamMethod();
private static volatile io.grpc.MethodDescriptor<testg.UserVo.User,
testg.UserVo.UserList> getSetUserStreamMethod;
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static io.grpc.MethodDescriptor<testg.UserVo.User,
testg.UserVo.UserList> getSetUserStreamMethod() {
io.grpc.MethodDescriptor<testg.UserVo.User, testg.UserVo.UserList> getSetUserStreamMethod;
if ((getSetUserStreamMethod = UserServerGrpc.getSetUserStreamMethod) == null) {
synchronized (UserServerGrpc.class) {
if ((getSetUserStreamMethod = UserServerGrpc.getSetUserStreamMethod) == null) {
UserServerGrpc.getSetUserStreamMethod = getSetUserStreamMethod =
io.grpc.MethodDescriptor.<testg.UserVo.User, testg.UserVo.UserList>newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.CLIENT_STREAMING)
.setFullMethodName(generateFullMethodName(
"testg.UserServer", "SetUserStream"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
testg.UserVo.User.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
testg.UserVo.UserList.getDefaultInstance()))
.setSchemaDescriptor(new UserServerMethodDescriptorSupplier("SetUserStream"))
.build();
}
}
}
return getSetUserStreamMethod;
}
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
@java.lang.Deprecated // Use {@link #getChatMethod()} instead.
public static final io.grpc.MethodDescriptor<testg.UserVo.User,
testg.UserVo.User> METHOD_CHAT = getChatMethod();
private static volatile io.grpc.MethodDescriptor<testg.UserVo.User,
testg.UserVo.User> getChatMethod;
@io.grpc.ExperimentalApi("https://github.com/grpc/grpc-java/issues/1901")
public static io.grpc.MethodDescriptor<testg.UserVo.User,
testg.UserVo.User> getChatMethod() {
io.grpc.MethodDescriptor<testg.UserVo.User, testg.UserVo.User> getChatMethod;
if ((getChatMethod = UserServerGrpc.getChatMethod) == null) {
synchronized (UserServerGrpc.class) {
if ((getChatMethod = UserServerGrpc.getChatMethod) == null) {
UserServerGrpc.getChatMethod = getChatMethod =
io.grpc.MethodDescriptor.<testg.UserVo.User, testg.UserVo.User>newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(generateFullMethodName(
"testg.UserServer", "Chat"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
testg.UserVo.User.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
testg.UserVo.User.getDefaultInstance()))
.setSchemaDescriptor(new UserServerMethodDescriptorSupplier("Chat"))
.build();
}
}
}
return getChatMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static UserServerStub newStub(io.grpc.Channel channel) {
return new UserServerStub(channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static UserServerBlockingStub newBlockingStub(
io.grpc.Channel channel) {
return new UserServerBlockingStub(channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static UserServerFutureStub newFutureStub(
io.grpc.Channel channel) {
return new UserServerFutureStub(channel);
}
/**
*/
public static abstract class UserServerImplBase implements io.grpc.BindableService {
/**
*/
public void getUserById(testg.UserVo.User request,
io.grpc.stub.StreamObserver<testg.UserVo.User> responseObserver) {
asyncUnimplementedUnaryCall(getGetUserByIdMethod(), responseObserver);
}
/**
*/
public void getList(testg.UserVo.Empty request,
io.grpc.stub.StreamObserver<testg.UserVo.UserList> responseObserver) {
asyncUnimplementedUnaryCall(getGetListMethod(), responseObserver);
}
/**
*/
public void getListStream(testg.UserVo.Empty request,
io.grpc.stub.StreamObserver<testg.UserVo.User> responseObserver) {
asyncUnimplementedUnaryCall(getGetListStreamMethod(), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver<testg.UserVo.User> setUserStream(
io.grpc.stub.StreamObserver<testg.UserVo.UserList> responseObserver) {
return asyncUnimplementedStreamingCall(getSetUserStreamMethod(), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver<testg.UserVo.User> chat(
io.grpc.stub.StreamObserver<testg.UserVo.User> responseObserver) {
return asyncUnimplementedStreamingCall(getChatMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getGetUserByIdMethod(),
asyncUnaryCall(
new MethodHandlers<
testg.UserVo.User,
testg.UserVo.User>(
this, METHODID_GET_USER_BY_ID)))
.addMethod(
getGetListMethod(),
asyncUnaryCall(
new MethodHandlers<
testg.UserVo.Empty,
testg.UserVo.UserList>(
this, METHODID_GET_LIST)))
.addMethod(
getGetListStreamMethod(),
asyncServerStreamingCall(
new MethodHandlers<
testg.UserVo.Empty,
testg.UserVo.User>(
this, METHODID_GET_LIST_STREAM)))
.addMethod(
getSetUserStreamMethod(),
asyncClientStreamingCall(
new MethodHandlers<
testg.UserVo.User,
testg.UserVo.UserList>(
this, METHODID_SET_USER_STREAM)))
.addMethod(
getChatMethod(),
asyncBidiStreamingCall(
new MethodHandlers<
testg.UserVo.User,
testg.UserVo.User>(
this, METHODID_CHAT)))
.build();
}
}
/**
*/
public static final class UserServerStub extends io.grpc.stub.AbstractStub<UserServerStub> {
private UserServerStub(io.grpc.Channel channel) {
super(channel);
}
private UserServerStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected UserServerStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new UserServerStub(channel, callOptions);
}
/**
*/
public void getUserById(testg.UserVo.User request,
io.grpc.stub.StreamObserver<testg.UserVo.User> responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetUserByIdMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getList(testg.UserVo.Empty request,
io.grpc.stub.StreamObserver<testg.UserVo.UserList> responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetListMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getListStream(testg.UserVo.Empty request,
io.grpc.stub.StreamObserver<testg.UserVo.User> responseObserver) {
asyncServerStreamingCall(
getChannel().newCall(getGetListStreamMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver<testg.UserVo.User> setUserStream(
io.grpc.stub.StreamObserver<testg.UserVo.UserList> responseObserver) {
return asyncClientStreamingCall(
getChannel().newCall(getSetUserStreamMethod(), getCallOptions()), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver<testg.UserVo.User> chat(
io.grpc.stub.StreamObserver<testg.UserVo.User> responseObserver) {
return asyncBidiStreamingCall(
getChannel().newCall(getChatMethod(), getCallOptions()), responseObserver);
}
}
/**
*/
public static final class UserServerBlockingStub extends io.grpc.stub.AbstractStub<UserServerBlockingStub> {
private UserServerBlockingStub(io.grpc.Channel channel) {
super(channel);
}
private UserServerBlockingStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected UserServerBlockingStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new UserServerBlockingStub(channel, callOptions);
}
/**
*/
public testg.UserVo.User getUserById(testg.UserVo.User request) {
return blockingUnaryCall(
getChannel(), getGetUserByIdMethod(), getCallOptions(), request);
}
/**
*/
public testg.UserVo.UserList getList(testg.UserVo.Empty request) {
return blockingUnaryCall(
getChannel(), getGetListMethod(), getCallOptions(), request);
}
/**
*/
public java.util.Iterator<testg.UserVo.User> getListStream(
testg.UserVo.Empty request) {
return blockingServerStreamingCall(
getChannel(), getGetListStreamMethod(), getCallOptions(), request);
}
}
/**
*/
public static final class UserServerFutureStub extends io.grpc.stub.AbstractStub<UserServerFutureStub> {
private UserServerFutureStub(io.grpc.Channel channel) {
super(channel);
}
private UserServerFutureStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected UserServerFutureStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new UserServerFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture<testg.UserVo.User> getUserById(
testg.UserVo.User request) {
return futureUnaryCall(
getChannel().newCall(getGetUserByIdMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture<testg.UserVo.UserList> getList(
testg.UserVo.Empty request) {
return futureUnaryCall(
getChannel().newCall(getGetListMethod(), getCallOptions()), request);
}
}
private static final int METHODID_GET_USER_BY_ID = 0;
private static final int METHODID_GET_LIST = 1;
private static final int METHODID_GET_LIST_STREAM = 2;
private static final int METHODID_SET_USER_STREAM = 3;
private static final int METHODID_CHAT = 4;
private static final class MethodHandlers<Req, Resp> implements
io.grpc.stub.ServerCalls.UnaryMethod<Req, Resp>,
io.grpc.stub.ServerCalls.ServerStreamingMethod<Req, Resp>,
io.grpc.stub.ServerCalls.ClientStreamingMethod<Req, Resp>,
io.grpc.stub.ServerCalls.BidiStreamingMethod<Req, Resp> {
private final UserServerImplBase serviceImpl;
private final int methodId;
MethodHandlers(UserServerImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver<Resp> responseObserver) {
switch (methodId) {
case METHODID_GET_USER_BY_ID:
serviceImpl.getUserById((testg.UserVo.User) request,
(io.grpc.stub.StreamObserver<testg.UserVo.User>) responseObserver);
break;
case METHODID_GET_LIST:
serviceImpl.getList((testg.UserVo.Empty) request,
(io.grpc.stub.StreamObserver<testg.UserVo.UserList>) responseObserver);
break;
case METHODID_GET_LIST_STREAM:
serviceImpl.getListStream((testg.UserVo.Empty) request,
(io.grpc.stub.StreamObserver<testg.UserVo.User>) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver<Req> invoke(
io.grpc.stub.StreamObserver<Resp> responseObserver) {
switch (methodId) {
case METHODID_SET_USER_STREAM:
return (io.grpc.stub.StreamObserver<Req>) serviceImpl.setUserStream(
(io.grpc.stub.StreamObserver<testg.UserVo.UserList>) responseObserver);
case METHODID_CHAT:
return (io.grpc.stub.StreamObserver<Req>) serviceImpl.chat(
(io.grpc.stub.StreamObserver<testg.UserVo.User>) responseObserver);
default:
throw new AssertionError();
}
}
}
private static abstract class UserServerBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
UserServerBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return testg.UserServerOuterClass.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("UserServer");
}
}
private static final class UserServerFileDescriptorSupplier
extends UserServerBaseDescriptorSupplier {
UserServerFileDescriptorSupplier() {}
}
private static final class UserServerMethodDescriptorSupplier
extends UserServerBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
UserServerMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (UserServerGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new UserServerFileDescriptorSupplier())
.addMethod(getGetUserByIdMethod())
.addMethod(getGetListMethod())
.addMethod(getGetListStreamMethod())
.addMethod(getSetUserStreamMethod())
.addMethod(getChatMethod())
.build();
}
}
}
return result;
}
}
<file_sep>/README.md
# grpcserver
这是一个grpc练习的服务端
大家可以到博客看详情
grpc基本实践(一)
https://blog.csdn.net/aixinaxc/article/details/79939555
grpc基础实践(二)
https://blog.csdn.net/aixinaxc/article/details/79948602
| 05a689a173f8d6746271bd3be3c23c5e269517f2 | [
"Markdown",
"Java",
"Go"
] | 3 | Go | ChinaMCat/grpcserver | 061672d395fe65332e7fe1cfe639224ed301f277 | 95a4b19c084131282917c70532ce2fe828dc6acf |
refs/heads/master | <repo_name>nya429/Sibi<file_sep>/app/Components/Yelp.js
let accessToken = '';
const CLIENT_ID = 'Bi10Mnj4rw_Bg7F5j6naVA';
const CLIENT_SECRET = '<KEY>';
let authUrl = `https://cors-anywhere.herokuapp.com/https://api.yelp.com/oauth2/token?grant_type=client_credentials&client_id=${CLIENT_ID}&client_secret=${CLIENT_SECRET}`;
let crd = {
latitude:0,
longitude:0
};
const Yelp = {
search(term,location,sortBy='best_match') {
if (!location) {
return new Promise((resolve,reject) => {
navigator.geolocation.getCurrentPosition(pos => {
crd.latitude = pos.coords.latitude;
crd.longitude = pos.coords.longitude;
resolve(crd)
});
}).then(crd => {
let url = `https://cors-anywhere.herokuapp.com/https://api.yelp.com/v3/businesses/search?limit=5&term=${term}&latitude=${crd.latitude}&longitude=${crd.longitude}&sort_by=${sortBy}`;
return this.getBusiness(url)
})
} else {
let url = `https://cors-anywhere.herokuapp.com/https://api.yelp.com/v3/businesses/search?limit=5&term=${term}&location=${location}&sort_by=${sortBy}`;
return this.getBusiness(url);
}
},
getBusiness(url) {
console.log('getBusiness');
return this.getAccessToken().then(() => {
return fetch(url,{
headers:{'Authorization':`Bearer ${accessToken}`,'origin':'https://api.yelp.com/v3/businesses/search'}
});
}).then(response => response.json()).then(jsonResponse => {
if(!jsonResponse.businesses) {
return;
} else {
return jsonResponse.businesses.map(business => ({
id:business.id,
imageSrc:business.image_url,
name:business.name,
address:business.location.address1,
city:business.location.city,
state:business.location.state,
zipCode:business.location.zip_code,
category:business.categories,
rating:business.rating,
reviewCount:business.review_count
}));
}
}).catch(err => console.error(err));
},
getAccessToken() {
if (accessToken) {
return new Promise(resolve => resolve(accessToken));
}
return fetch(authUrl,{
method:'POST',
headers:{
'origin':'https://api.yelp.com/' //must have it here
},
}).then(response => response.json()).then(
jsonResponse =>
accessToken = jsonResponse.access_token).catch(err => console.error(err));
}
}
export default Yelp;
<file_sep>/index.android.js
/**
* Sample React Native App
* https://github.com/facebook/react-native
* @flow
*/
import React, { Component } from 'react';
import SearchBar from './app/Components/SearchBar';
import BusinessList from './app/Components/BusinessList';
import Yelp from './app/Components/Yelp';
import Styles from './app/Styles/Main';
import {
AppRegistry,
StyleSheet,
View,
Image,
Alert,
Dimensions,
ScrollView,
ListView,
ToastAndroid,
Platform,
} from 'react-native';
/**
*/
export default class AwesomeProject extends Component {
constructor(props) {
super(props);
const dataSource = new ListView.DataSource({
rowHasChanged: (row1,row2) => row1 !== row2
});
this.state = {isLoaded:null,
businesses:dataSource,
loadingtext:'',
};
this._search = this._search.bind(this);
//Used to test fetch function
this.getmovie = function() {
return fetch('https://facebook.github.io/react-native/movies.json').then(response => response.json()).then(responseJson => responseJson.movies).catch( error => console.error(error));
}
}
handleSearchExpression() {
ToastAndroid.show('Nothing Found',ToastAndroid.SHORT);
this.setState({
isLoaded:false,
});
}
loadingTime() {
let loadExpression = 5000;
this.timeout = setTimeout(() => this.handleSearchExpression(), loadExpression);
}
_search(term,place) {
this.setState({isLoaded:true});
this.loadingTime();
Yelp.search(term,place).then(businesses => {
console.log('DEBUG andoird then')
console.log(businesses);
this.setState({
businesses:this.state.businesses.cloneWithRows(businesses),
isLoaded:false,
});
});
}
componentWillMount() {
if(Platform.OS === 'android') {
ToastAndroid.showWithGravity('Android Yelp',ToastAndroid.SHORT,ToastAndroid.CENTER);
}
}
componentWillUpdate(nextProps,nextState) {
if(this.state.businesses === nextState.businesses) {
}
}
render() {
return (
<View style={Styles.container}>
<Image style={style.backgroundImage} source={require('./img/background.jpg')} >
<View style={Styles.content}>
<SearchBar onTextInput={this._textInput} onPress={this._search} />
<BusinessList isLoaded={this.state.isLoaded} dataSource={this.state.businesses} />
</View >
</ Image>
</View>
);
}
}
const functions = {
ex: {
width: Dimensions.get('window').width,
height: Dimensions.get('window').height
},
}
const style = StyleSheet.create({
backgroundImage: {
flex: 1,
height:functions.ex.height,
},
})
AppRegistry.registerComponent('AwesomeProject', () => AwesomeProject);
<file_sep>/app/Components/BusinessList.js
import React, { Component } from 'react';
import Styles from '../Styles/Main';
import {
ListView,
Text,
View,
Image,
} from 'react-native';
class BusinessList extends Component {
constructor(props) {
super(props);
this.state = {
loadingText:'',
}
this.count = 12;
}
_scorllText (text) {
if(this.count < text.length) {
this.count ++;
} else {
this.count = 12;
}
this.setState({loadingText:text.slice(0,this.count)});
}
_renderLoading() {
return (<View style={Styles.loading}>
<Text style={Styles.loadingText}>{this.state.loadingText}</Text>
</View>);
}
componentWillUpdate(nextProps,nextState) {
if(nextProps.isLoaded === false) {
clearInterval(this.interval);
this.interval = '';
} else if(!this.interval ) {
let text = `Where to eat .... `;
this.count = 12;
this.interval = setInterval(() => this._scorllText(text),100);
}
}
_renderRow(business) {
return (
<View style={Styles.business}>
<Image style={Styles.businessImage} source={{'uri':business.imageSrc}} />
<View style={Styles.businessContent}>
<Text style={Styles.businessName}>{business.name}</Text>
<Text style={Styles.businessRating}> <Text style={{fontSize:23,color:'rgb(255, 187, 0)'}}>{business.rating}</Text> | {business.reviewCount} reviews</Text>
<Text style={Styles.businessAddress}>{business.address}{'\n'}{business.city}, {business.state}{'\n'}{business.zipCode} </Text>
</View>
</View>
);
}
_renderviewList() {
if(this.props.isLoaded) {
return this._renderLoading();
} else {
return (<ListView
style= {Styles.businessListView}
enableEmptySections={true}
dataSource={this.props.dataSource}
renderRow={business => this._renderRow(business)}
/>);
}
}
render() {
return this._renderviewList();
}
}
export default BusinessList;
<file_sep>/app/Styles/Main.js
import { StyleSheet,Dimensions } from 'react-native';
const Styles = StyleSheet.create({
container: {
flex: 1,
flexDirection:'row',
justifyContent:'center',
alignItems:'center',
},
content:{
height:'100%',
flexDirection:'column',
justifyContent:'center',
alignItems:'center',
},
instructions: {
marginTop:-60,
marginLeft:240,
textShadowColor:'rgb(163, 150, 97)',
textShadowOffset:{height:2,width:1},
color:'rgba(35, 33, 27,0.9)',
textAlign: 'center',
fontFamily: 'Helvetica Neue',
fontSize:20,
letterSpacing: 2,
lineHeight:23,
fontWeight: 'bold',
},
inputContainer: {
flexDirection:'row',
marginTop:40,
},
placeholderStyle:{
fontFamily: "AnotherFont",
borderColor: 'rgba(255,255,255,0.1)'
},
textInput:{
marginVertical:10,
height:50,
width:180,
fontSize:16,
marginHorizontal:10,
borderWidth:1,
borderColor:'rgb(255, 187, 0)',
fontWeight:'bold',
color:"rgb(224, 206, 74)",
backgroundColor:'rgba(36, 40, 33, 0.78)',
},
touchableOpacityText:{
alignSelf:'center',
fontFamily:'Helvetica',
marginVertical:25,
fontSize:33,
color:'rgb(255,255,255)',
},
touchableOpacityView: {
margin:100,
shadowOffset:{height:5,width:6},
shadowOpacity:0.6,
shadowRadius:2,
shadowColor:'rgba(255, 255, 255, 0.25)',
borderRadius:100,
margin:1.5,
width:97,
height:97,
backgroundColor:'rgba(135, 113, 66, 0.9)',
},
touchableOpacity: {
marginTop:20,
},
businessListView:{
},
business: {
backgroundColor:'rgba(36, 40, 33, 0.78)',
marginVertical:4,
width:380,
height:180,
borderColor:'rgb(255, 187, 0)',
borderWidth:1,
flexDirection:'row',
},
businessImage: {
height:170,
width:170,
margin:5
},
businesContent:{
marginLeft:10,
fontSize:14,
color:'white'
},
businessName: {
marginTop:10,
marginLeft:10,
fontSize:22,
fontWeight:'bold',
color:'rgb(249, 233, 112)',
},
businessRating: {
marginLeft:15,
marginTop:10,
fontSize:18,
color:'rgb(249, 233, 112)',
},
businessAddress: {
color:'rgba(249, 233, 112, 0.7)',
marginTop:5,
marginLeft:20,
fontSize:18,
},
loading: {
height:461,
width:200,
alignItems:'flex-start',
},
loadingText: {
fontSize:23,
color:'rgb(249, 233, 112)',
marginTop:50,
},
});
export default Styles;
| 276432656c53194ae702d85a7e18a041292ccb60 | [
"JavaScript"
] | 4 | JavaScript | nya429/Sibi | f0d3e43d70e740d650e052c40dea5677aa676861 | 2471161116f1e443a9749056132281f2f5372a54 |
refs/heads/master | <file_sep>const assert = require("assert");
describe ("socialbot interceptor", () => {
const interceptor = require("../index");
it("should exist", () => {
assert(interceptor !== undefined);
});
it("should return a function", () => {
assert(typeof interceptor === "function");
});
describe("when responding", () => {
let
called,
req,
res,
next;
beforeEach(() => {
called = false,
req = { get: () => "Twitterbot" },
res = { send: () => null },
next = () => null;
});
it("should check for request to be from a bot", () => {
// success case
interceptor(null, () => {
called = true;
})(req, res, next);
assert(called === true);
called = false;
req = { get: () => `Mozilla/5.0 (iPad; U; CPU OS 3_2_1 like Mac OS X; en-us) AppleWebKit/531.21.10 (KHTML, like Gecko) Mobile/7B405` };
// failure case
interceptor(null, () => {
called = true;
})(req, res, next);
assert(called === false);
});
it("should not proceed by default", () => {
let _next = () => called = true;
interceptor({}, (req, res, _next) => {
called = false;
})(req, res, next);
assert(called === false);
});
it("should proceed if set", () => {
interceptor({ shouldProceed: true }, (req, res, () => called = true))(req, res, next);
assert(called === true);
});
});
});
<file_sep>/*
* redirect requests from social media
* to different renderer
*/
function Render(cfg, cb = () => null) {
let
callback = cb;
agents = [
"Twitterbot",
"facebookexternalhit",
];
config = {
shouldProceed: false,
shouldWait: false,
};
// overwrite
overwrite(cfg);
return function (req, res, next) {
let
headers = req.get("user-agent"),
match = false,
proceed = null;
agents.forEach(agent => {
if (headers.match(agent)) {
match = true;
}
});
if (match === true) {
proceed = cb(req, res, next);
} else {
next();
}
// if go to next route handler
if (config.shouldProceed) {
// if async
if (config.shouldWait) {
proceed.then(() => next());
}
// just proceed
else {
next();
}
}
}
function overwrite() {
if (cfg === null) return;
Object.keys(cfg).forEach(key => {
config[key] = cfg[key];
});
}
}
module.exports = Render;
<file_sep># socialbots-interceptor
An ExpressJS middleware to intercept requests from social media service crawlers (like Twitter, Facebook) and allow defining a custom handler.
## Usage
```javascript
const Interceptor = require("socialbots-interceptor");
// ...
app.use(Interceptor(cfg, (req, res, next) => {
// ...
res.status(404).send("Not found");
}));
```
## Options
* `shouldProceed` (boolean, default `false`) makes the interceptor non-blocking
* `shouldWait` (boolean, default `false`) waits on a promise from the given callback to proceed
*Note* Enabling either of them is not recommended. Your handler should completely handle and respond to the request, then quit.
## Supports
Currently supports:
* Twitter (`Twitterbot`)
* Facebook (`facebookexternalhit`)
| f149bc62aae2f212ee94da2ce7f4fdfb7091c82c | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | adityavm/socialbots-interceptor | 21691912079b177c7e6e666fb6f826da021e750c | d227f426f468f8609ad8369e859044c7c35f3a02 |
refs/heads/master | <file_sep>// const charactersAPI = new APIHandler('https://minions-api.herokuapp.com/characters');
// window.addEventListener('load', () => {
// document.getElementById('fetch-all').addEventListener('click', function (event) {
// });
// document.getElementById('fetch-one').addEventListener('click', function (event) {
// });
// document.getElementById('delete-one').addEventListener('click', function (event) {
// });
// document.getElementById('edit-character-form').addEventListener('submit', function (event) {
// });
// document.getElementById('new-character-form').addEventListener('submit', function (event) {
// });
// Esto es basura });
const buttonSearchOne = document.getElementById("fetch-one")
const inputs = document.getElementsByTagName('input')
buttonSearchOne.onclick = e => {
e.preventDefault()
const id = document.getElementById('idOne').value
axios.get(`https://minions-api.herokuapp.com/characters/${id}`)
.then(response => {
console.log(response.data)
const { name, occupation, cartoon, weapon } = response.data
console.log(inputs[0])
inputs[0].value = name
inputs[1].value = occupation
inputs[2].value = cartoon
inputs[3].value = weapon
})
.catch(err => console.log('error al recobrar 1 personaje', err))
}
const buttonDelete = document.getElementById("delete-one")
buttonDelete.onclick = e => {
e.preventDefault()
const id = document.getElementsByName("character-id-delete")[0].value
console.log(id)
axios.delete(`https://minions-api.herokuapp.com/characters/${id}`, { name, occupation, cartoon, weapon })
}
const buttonCreate = document.getElementById('send-data')
buttonCreate.onclick = e => {
e.preventDefault()
const characterInfo = {
name: inputs[6].value,
occupation: inputs[7].value,
weapon: inputs[8].value,
cartoon: inputs[9].checked
}
axios.post(`https://minions-api.herokuapp.com/characters`, characterInfo)
.then(response => {
const { name, occupation, weapon, cartoon } = response.data
})
.catch(err => console.log('error al crear personaje', err))
}
| 9bdba5d4168bafc47a202c2373f4c5e328cfdd95 | [
"JavaScript"
] | 1 | JavaScript | MarvinSequera/lab-ajax-crud-characters | 890480258f30775832fc73c515b8305c29017ed3 | b31e28e2f8b4256505138300fb82c754c457e3c1 |
refs/heads/main | <file_sep>import { combineReducers, configureStore } from '@reduxjs/toolkit'
import itineraryReducer from '../lib/slices/itinerarySlice'
import searchReducer from '../lib/slices/searchSlice'
const rootReducer = combineReducers({
itineraries: itineraryReducer,
search: searchReducer
})
export type CoreState = ReturnType<typeof rootReducer>
const store = configureStore({
reducer: rootReducer,
devTools: true
})
export default store
export type RootState = ReturnType<typeof store.getState>
export type AppDispatch = typeof store.dispatch
<file_sep>module.exports = {
assetPrefix: '/trail-assignment/',
basePath: '/trail-assignment'
}
<file_sep>import { createAsyncThunk, createSlice, PayloadAction } from '@reduxjs/toolkit'
import { CoreState } from '../../store'
type SearchState = {
search: {
term: string
reverse: boolean
address: {
name: string
coords: {
latitude: number
longitude: number
}
}
}
}
interface AddressSearchArgs {
term?: string
coords?: {
latitude: number
longitude: number
}
}
export const addressSearch = createAsyncThunk(
'search/addressSearch',
async ({
term,
coords
}: AddressSearchArgs): Promise<SearchState['search']['address']> => {
let res
if (term && coords) {
res = fetch(
`https://api.digitransit.fi/geocoding/v1/search?text=${term}&size=1&focus.point.lat=${coords.latitude}&focus.point.lon=${coords.longitude}`
)
} else if (term) {
res = fetch(
`https://api.digitransit.fi/geocoding/v1/search?text=${term}&size=1`
)
} else if (coords) {
res = fetch(
`https://api.digitransit.fi/geocoding/v1/reverse?point.lat=${coords.latitude}&point.lon=${coords.longitude}&size=1`
)
} else {
throw new Error('Nothing to search with')
}
const json = await (await res).json()
return {
name: json.features[0].properties.label,
coords: {
latitude: json.features[0].geometry.coordinates[1],
longitude: json.features[0].geometry.coordinates[0]
}
}
}
)
const initialState: SearchState = {
search: {
term: '',
reverse: false,
address: {
name: '',
coords: {
latitude: 0,
longitude: 0
}
}
}
}
const searchSlice = createSlice({
name: 'search',
initialState,
reducers: {
updateTerm: (state, action: PayloadAction<string>) => {
state.search.term = action.payload
},
updateReverse: (state, action: PayloadAction<boolean>) => {
state.search.reverse = action.payload
}
},
extraReducers: (builder) => {
builder.addCase(
addressSearch.fulfilled,
(state, action: PayloadAction<SearchState['search']['address']>) => {
state.search.address = action.payload
}
)
}
})
export const selectSearch = (state: CoreState) => state.search
export const { updateTerm, updateReverse } = searchSlice.actions
export default searchSlice.reducer
<file_sep>import { createAsyncThunk, createSlice, PayloadAction } from '@reduxjs/toolkit'
import { CoreState } from '../../store'
import { request, gql } from 'graphql-request'
interface SchedulesQueryArgs {
latitude: number
longitude: number
reverse?: boolean
}
const SchedulesQuery = ({
latitude,
longitude,
reverse
}: SchedulesQueryArgs) => {
// prettier-ignore
const from = `{ lat: ${reverse ? 60.166947 : latitude}, lon: ${reverse ? 24.921601 : longitude } }`
// prettier-ignore
const to = `{ lat: ${reverse ? latitude : 60.166947}, lon: ${ reverse ? longitude : 24.921601 } }`
return gql`
{
plan(
from: ${from}
to: ${to}
numItineraries: 10
) {
itineraries {
startTime
legs {
mode
startTime
endTime
from {
lat
lon
name
stop {
code
name
gtfsId
stoptimesForPatterns(omitNonPickups: true, timeRange: 86400) {
pattern {
code
}
stoptimes {
scheduledDeparture
}
}
}
}
to {
lat
lon
name
stop {
patterns {
code
}
}
}
trip {
gtfsId
pattern {
code
name
headsign
}
tripHeadsign
}
}
}
}
}
`
}
export const fetchItineraries = createAsyncThunk(
'schedule/fetchSchedule',
async ({
latitude,
longitude,
reverse
}: SchedulesQueryArgs): Promise<ItineraryState> => {
const { plan } = await request(
'https://api.digitransit.fi/routing/v1/routers/hsl/index/graphql',
SchedulesQuery({ latitude, longitude, reverse })
)
return plan
}
)
type ItineraryState = {
itineraries: {
startTime: number
legs: {
startTime: number
endTime: number
mode: 'WALK' | 'BUS' | 'SUBWAY' | 'RAIL' | 'TRAM' | 'FERRY'
from: {
lat: number
long: number
name: string
stop: {
code: string
name: string
gtfsId: string
stopTimesForPatterns: {
pattern: {
code: string
}
stoptimes: {
scheduledDeparture: number
}[]
}[]
} | null
}
to: {
lat: number
long: number
name: string
stop: {
patterns: {
code: string
}[]
}
}
trip: {
gtfsId: string
tripHeadsign: string
pattern: {
code: string
name: string
}
} | null
}[]
}[]
}
const initialState: ItineraryState = {
itineraries: []
}
const itinerarySlice = createSlice({
name: 'itineraries',
initialState,
reducers: {
update: (state, action: PayloadAction<ItineraryState>) => {
state.itineraries = action.payload.itineraries
}
},
extraReducers: (builder) => {
builder.addCase(
fetchItineraries.fulfilled,
(state, action: PayloadAction<ItineraryState>) => {
state.itineraries = action.payload.itineraries
}
)
}
})
export const selectItinerary = (state: CoreState) => state.itineraries
export const { update } = itinerarySlice.actions
export default itinerarySlice.reducer
<file_sep># Trail Systems Frontend Developer Assignment
This is a solution to a recruitment assignment by Trail Systems.
## Stack
- TypeScript
- Next.js
- Redux
- GraphQL
- Material-UI
## About
The task was to build an online public transportation timetable for Maria 01 using the HSL open data interface.
| 56de968352ee4fc7f811eff61c5262a31ddb6ff6 | [
"JavaScript",
"TypeScript",
"Markdown"
] | 5 | TypeScript | Topias-R/trail-assignment | 845710996fdeba1ad24a7f1f3de5a8c3b1165ade | f1a001b0da378d8493679c722d5e70f12eb8f9f5 |
refs/heads/master | <file_sep><?php
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Auth::routes();
Route::get('/', 'HomeController@index')->name('home');
Route::prefix('home')->group(function(){
Route::get('/', 'HomeController@index')->name('home');
Route::get('/support', 'HomeController@index')->name('support');
Route::get('/update-core', 'HomeController@index')->name('home/update-core');
Route::get('/documentation', 'HomeController@index')->name('home/documentation');
});
Route::prefix('users')->group(function(){
Route::get('/', 'UserController@index')->name('users');
Route::get('/users-data', 'UserController@getUsersData')->name('users-data');
Route::get('/create', 'UserController@create')->name('users/create');
Route::post('/create', 'UserController@store')->name('users/create');
Route::get('/edit/{id}', 'UserController@edit')->name('users/edit');
Route::post('/edit/{id}', 'UserController@update')->name('users/edit');
Route::get('/show/{id}', 'UserController@show')->name('users/show');
Route::get('/delete/{id}', 'UserController@destroy')->name('users/delete');
Route::get('users/profile', 'UserController@editProfile')->name('users/profile');
});
Route::prefix('courses')->group(function(){
Route::get('/', 'CourseController@index')->name('courses');
Route::get('/create', 'CourseController@index')->name('courses/create');
Route::get('/enroll', 'CourseController@index')->name('courses/enroll');
Route::get('/enroll', 'CourseController@index')->name('courses/enroll');
});
Route::prefix('classes')->group(function(){
Route::get('/', 'ClassController@index')->name('classes');
Route::get('/create', 'ClassController@index')->name('classes/create');
Route::get('/show/{id}', 'ClassController@index')->name('classes/show');
Route::get('/edit/{id}', 'ClassController@index')->name('classes/eidt');
});
<file_sep><?php
namespace App\Http\Controllers;
use Request as DataRequest;
use Illuminate\Http\Request;
use Illuminate\Http\Response;
use App\User;
use Validator;
use Carbon\Carbon;
use Yajra\DataTables\Facades\DataTables;
use App\Http\Controllers\Controller;
use Auth;
class UserController extends Controller{
/**
* Create a new controller instance.
*
* @return void
*/
public function __construct()
{
$this->middleware('auth');
}
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
return view('pages.users.index');
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('pages.users.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$this->validate($request, [
'first_name' => 'required|string|max:255',
'last_name' => 'required|string|max:255',
'user_type' => 'required|string|max:255',
'email' => 'required|string|email|max:255|unique:users',
'password' => '<PASSWORD>',
]);
$user = User::create([
'first_name' => $request->first_name,
'last_name' =>$request->last_name,
'email' =>$request->email,
'password'=><PASSWORD>($request->password),
'status'=> false,
'type'=> $request->user_type,
'activation_key'=>'',
'last_login'=>Carbon::now(),
]);
if ($request->ajax()) {
return response()->json([
'success' => true,
'message' => "User was created successifully"
]);
}
return redirect()->route('users');
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function editProfile(){
$user = Auth::user();
return view('pages.users.profile', compact('user'));
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id){
$user = User::find($id);
if(DataRequest::ajax()){
$response = response()->json([
'id' => $user->id,
'first_name' => $user->first_name,
'last_name' => $user->last_name,
'email' => $user->email,
'type' => $user->type,
'status' => $user->status
]);
return $response;
}
return view('pages.users.edit', compact('user'));
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
$user = User::find($id);
if(DataRequest::ajax()){
$response = response()->json([
'id' => $user->id,
'first_name' => $user->first_name,
'last_name' => $user->last_name,
'email' => $user->email,
'type' => $user->type,
'status' => $user->status
]);
return $response;
}
return view('pages.users.show', compact('user'));
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id){
$user = User::find($id);
$user->first_name = $request->first_name;
$user->last_name = $request->last_name;
$user->save();
if ($request->ajax()) {
return response()->json([
'sucess' => true,
]);
}
return redirect()->route('users');
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id){
$user = User::find($id);
$user->delete();
}
/**
* Process datatables ajax request.
*
* @return \Illuminate\Http\JsonResponse
*/
public function getUsersData(){
$users = User::query();
return Datatables::of($users)
->addColumn('action', function ($user) {
return '<a href="javascript:void(0)" onclick="editRow('.$user->id.')" class="btn btn-xs btn-success"><i class="fa fa-pencil" aria-hidden="true"></i> Edit</a>
<a href="javascript:void(0)" data-id="row-' . $user->id . '" onclick="removeRow('.$user->id.')" class="btn btn-xs btn-danger"><i class="fa fa-trash" aria-hidden="true"></i> delete</a>';
})
->make(true);
}
}
<file_sep># srms-dashboard
Users dashboard
<file_sep><?php
class UsersTableSeeder extends UserSeeder{
protected $table = "users";
public function getData() {
$data = [
[
'first_name' =>'Otoman',
'last_name' =>'Nkomanya',
'email' => '<EMAIL>',
'password' => Hash::make('<PASSWORD>'),
'status' =>true,
'type'=>'admin',
'last_login'=>'2017-09-01',
'activation_key'=>'#$FTAHGBSBJSJJXLSAOJDNKUATFAIUGSOO+_%$%)'
],
[
'first_name' =>'Amon',
'last_name' =>'Bagoye',
'email' => '<EMAIL>',
'password' => Hash::make('<PASSWORD>'),
'status'=>'1',
'type'=>'student',
'last_login'=>'2017-09-01',
'activation_key'=>'#$FTAHGBSBJSJJXLSAOJDNKUATFAIUGSOO+_%$%)'
],
[
'first_name' =>'Joshua',
'last_name' =>'Mwambene',
'email' => '<EMAIL>',
'password' => Hash::make('<PASSWORD>'),
'status' =>true,
'type'=>'student',
'last_login'=>'2017-09-01',
'activation_key'=>'#$FTAHGBSBJSJJXLSAOJDNKUATFAIUGSOO+_%$%)'
],
[
'first_name' =>'Azori',
'last_name' =>'Bhilangoye',
'email' => '<EMAIL>',
'password' => Hash::make('<PASSWORD>'),
'status'=>'1',
'type'=>'student',
'last_login'=>'2017-09-01',
'activation_key'=>'#$FTAHGBSBJSJJXLSAOJDNKUATFAIUGSOO+_%$%)'
],
[
'first_name' =>'Festo',
'last_name' =>'Godfrey',
'email' => '<EMAIL>',
'password' => <PASSWORD>('<PASSWORD>'),
'status' =>true,
'type'=>'employee',
'last_login'=>'2017-09-01',
'activation_key'=>'#$FTAHGBSBJSJJXLSAOJDNKUATFAIUGSOO+_%$%)'
],
[
'first_name' =>'Zan',
'last_name' =>'Tejider',
'email' => '<EMAIL>',
'password' => <PASSWORD>('<PASSWORD>'),
'status'=>'1',
'type'=>'employee',
'last_login'=>'2017-09-01',
'activation_key'=>'#$FTAHGBSBJSJJXLSAOJDNKUATFAIUGSOO+_%$%)'
],
[
'first_name' =>'Kakozi',
'last_name' =>'Sadock',
'email' => '<EMAIL>',
'password' => <PASSWORD>('<PASSWORD>'),
'status' =>true,
'type'=>'admin',
'last_login'=>'2017-09-01',
'activation_key'=>'#$FTAHGBSBJSJJXLSAOJDNKUATFAIUGSOO+_%$%)'
],
[
'first_name' =>'Allen',
'last_name' =>'Joseph',
'email' => '<EMAIL>',
'password' => <PASSWORD>('<PASSWORD>'),
'status'=>'1',
'type'=>'student',
'last_login'=>'2017-09-01',
'activation_key'=>'#$FTAHGBSBJSJJXLSAOJDNKUATFAIUGSOO+_%$%)'
]
];
return $data;
}
}
| 2e4e07ba4004f852433088011f7c6bbaf3c7d42d | [
"Markdown",
"PHP"
] | 4 | PHP | otuman/srms | 8cbe1ee745499c7c861d4ad01a4e617f088be0cf | 2dbabd0d5e5351dde4474df620294a169debc963 |
refs/heads/master | <repo_name>alexandrego/VM<file_sep>/pag/home.php
<!DOCTYPE html>
<html lang="pt-br">
<div id="principal">
<img id="cameraHikvision" src="<?php echo $cameraHikvision ?>" />
<p id="textoCamera">
Fique tranquilo </br>
a gente fica de </br>
olho para você!
</p>
</div>
<!--Promoções e Destaques -->
<ul class="demo-2 effect">
<li id="produtos">
<h2 class="zero">Kit Elgin</h2>
<p class="zero">Kit completo de monitoramento Elgin.</p>
<p class="zero">R$ 1.000,00 em até 12 vezes.</p>
</li>
<li><img class="top" src="<?php echo $kitElgin ?>" /></li>
</ul>
<ul class="demo-2 effect">
<li>
<h2 class="zero">Lâmpadas espiãs</h2>
<p class="zero">Veja e ouço tudo
em qualquer ambiente sem ser notado.</p>
<p class="zero">R$ 275,00 em até 12 vezes.</p>
</li>
<li><img class="top" src="<?php echo $cameraespia ?>" /></li>
</ul>
<ul class="demo-2 effect">
<li>
<h2 class="zero">Portões Eletrônicos</h2>
<p class="zero">Confira toda nossa
linha de automatização.</p>
<p class="zero">R$ 000,00 em até 12 vezes.</p>
</li>
<li><img class="top" src="<?php echo $portao ?>" /></li>
</ul>
<ul class="demo-2 effect">
<li>
<h2 class="zero">Estabilizadores</h2>
<p class="zero">Tenha a proteção ideal
contra quedas de energia.</p>
<p class="zero">R$ 000,00 em até 12 vezes.</p>
</li>
<li><img class="top" src="<?php echo $estabilizador ?>" /></li>
</ul>
<ul class="demo-2 effect">
<li>
<h2 class="zero">Cerca Eletrica</h2>
<p class="zero">Kit completo de cercas eletricas.</p>
<p class="zero">R$ 000,00 em até 12 vezes.</p>
</li>
<li><img class="top" src="<?php echo $kitcerca ?>" /></li>
</ul>
<ul class="demo-2 effect">
<li>
<h2 class="zero">Camera Dome</h2>
<p class="zero">Que tal visualizar tudo
atráves das câmeras dome.</p>
<p class="zero">R$ 000,00 em até 12 vezes.</p>
</li>
<li><img class="top" src="<?php echo $cameradome ?>" /></li>
</ul>
<ul class="demo-2 effect">
<li>
<h2 class="zero">Cabos Trançados</h2>
<p class="zero">Possuimos cabos de rede, cabos de video, HDMI e mais.</p>
<p class="zero">Tudo em até 12 vezes.</p>
</li>
<li><img class="top" src="<?php echo $cabotrancado ?>" /></li>
</ul>
<ul class="demo-2 effect">
<li>
<h2 class="zero">Conectores</h2>
<p class="zero">Possuimos uma grande variedade de conectores.</p>
<p class="zero">Tudo em até 12 vezes.</p>
</li>
<li><img class="top" src="<?php echo $conectores ?>" /></li>
</ul>
<div class="clear"></div>
</br><hr></br>
<div id="diga">
<video id="apresentacaoElgin" src="<?php echo $elgin ?>" controls preload >
<p>Seu browser não é compatível, <a href="<?php echo $elgin ?>">baixe este vídeo em formato MP4</a></p>
</video>
<div id="textoelgin">
<h2 align="center">Gravadores de Vídeo Híbridos</h2>
</br></br></br>
<p>
Disponíveis em 4, 8 ou 16 canais de vídeo, possuem saídas para HDMI e VGA, além de
entradas para gravação de áudio.</br></br>
Suporte para câmera IP.</br></br>
Detecção de movimento, mascaramento ou perda de vídeo.</br></br>
Permitem gravação em AHD-MH, AHD-L, D1, HD1 e CIF.</br></br>
<a href="#">Saiba mais...</a>
</p>
</div>
</div>
</br><hr></br>
<div id="diga">
<h3 id="servicos" align="center">Nossos serviços</h3>
<img id="tecnicoCFTV" src="<?php echo $tecnicoCFTV ?>" />
<div id="textCFTV">
<p>
Pode ficar tranquilo, nossos técnicos passam por treinamentos e
são habilitados para executar nossos projetos do começo ao fim.
</br> </br>
Já possui um sistema de CFTV instalado?</br>
Fazemos manutenções preventivas e corretivas.</br></br>
Mantenha seu sistema sempre seguro e funcional.
</p>
</div>
<img id="tecnicoEletrico" src="<?php echo $tecnicoEletrico ?>" />
<div id="textEletrico">
<p>
Elaboramos projetos elétricos.</br></br>
1. Predial</br>
2. Residencial e</br>
3. Industrial.</br></br>
1. Baixa e</br>
2. Alta Tensão.
</p>
</div>
</div>
<div class="clear"></div>
<hr></br>
<file_sep>/estrutura.php
<!DOCTYPE html>
<html lang="pt-br">
<head>
<meta charset="UTF-8"/>
<link href="estilo.css" rel="stylesheet" type="text/css" />
<title><?php echo $titulo.' | '.ucwords($pagina) ?></title>
</head>
<body>
<div id="container"> <!--Conteudo do site!-->
<div id="header"> <!--Primeira header da pagina!-->
</div> <!--header-->
<div id="logo"> <!--Logo da pagina!-->
Aqui é o logo da empresa!
</div> <!--logo-->
<div id="corpo"> <!--Corpo do site!-->
<div id="conteudo"> <!--Conteudo do site!-->
Aqui é o conteudo do site!
</div> <!--conteudo-->
<div id="sidebar"> <!--Espaço propaganda!-->
Aqui é a sidebar do site!
</div><!--sidebar-->
<div class="clear"></div>
</div><!--corpo-->
<div id="menu"> <!--Menu da pagina!-->
<ul>
<li><a href="#">Empresa</a></li>
<li><a href="#">Contato</a></li>
</ul>
</div> <!--menu-->
<div id="footer"> <!--Rodapé do site!-->
Aqui é o rodapé do site!
</div><!--footer-->
</div><!--container-->
</body>
</html>
<file_sep>/index.php
<!DOCTYPE html>
<html lang="pt-br">
<?php
error_reporting(E_ALL ^ E_NOTICE);
require_once('variaveis.php');
$p = $_GET['p'];
if (empty($p)){
$pagina = 'home';
} else {
$pagina = $p;
}
if (!file_exists('pag/'.$pagina.'.php')) {
$pagina = 'Página não encontrada!';
}
include('header.php');
include('logo.php');
?>
<div id="conteudo">
<?php
if (file_exists('pag/'.$pagina.'.php')){
include("pag/$pagina.php");
} else {
echo "A página que você tentou acessar não existe!";
}
?>
</div><!-- conteudo -->
<?php
include('menu.php');
include('footer.php');
?>
<file_sep>/variaveis.php
<?php
$empresa = 'VM Tecnologia e Segurança';
$home = 'localhost/cursos/vm_1.0/';
$header = '';
$logoVM = 'img/logVP.png';
$autor = '<NAME>';
$rodape = 'Copyright @ ' .date('Y '). $empresa. ' - Todos os direitos reservados!';
$titulo = 'VM Tecnologia e Segurança';
$descricao = 'VM Tecnologia, Segurança, Produtos e Serviços!';
$keywords = '#';
$cameraHikvision = 'img/camera.png';
$kitHikvision = 'img/kitHikvision.jpg';
$cameraespia = 'img/cameraespia.jpg';
$portao = 'img/portao.jpg';
$estabilizador = 'img/estabilizador.jpg';
$sirene = 'img/sirene.jpg';
$kitcerca = 'img/kitcerca.jpg';
$cameradome = 'img/cameradome.jpg';
$cabotrancado = 'img/cabotrancado.jpg';
$conectores = 'img/conectores.jpg';
$elgin = 'video/elgin.mp4';
$kitElgin = 'img/kitElgin.jpg';
$tecnicoCFTV = 'img/tecnicoCFTV.jpg';
$tecnicoEletrico = 'img/tecnicoEletrico.jpg';
?>
<file_sep>/jquery/jquery.destaques.js
/**
* Escopo de compatibilidade do jQuery
*/
(function($){
$(document).ready(function() {
// Cria uma div.paginas que receberá os paginadores
var div = $('<div></div>').addClass('paginas');
// Insere a div criada antes da lista de destaques
$('#blocoDestaques ul').before(div);
/**
* Roda o jQuery Cycle na lista (ul) que está
* dentro do bloco de destaques (#blocoDestaques)
*/
$('#blocoDestaques ul').cycle({
pager: 'div.paginas', // Paginadores
pause: true, // Pausa ao passar o mouse sobre ele?
pauseOnPagerHover: true // Pausa ao passar o mouse sobre as páginas?
});
});
})(jQuery);<file_sep>/header.php
<!DOCTYPE html>
<html lang="pt-br">
<head>
<meta charset="UTF-8"/>
<meta name="description" content="<?php echo $descricao?>" />
<meta name="keywords" content="<?php echo $keywords?>" />
<!--author - o nome do autor da página.-->
<meta name="autor" content="<?php echo $autor?>" />
<!-- Ícone da página -->
<link rel="shortcut icon" href="favicon.ico" type="image/x-icon">
<link rel="icon" href="favicon.ico" type="image/x-icon">
<!-- Viewport informa ao dispositvo que a escala inicial do layout é equivalente ao tamanho do mesmo. -->
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Formatação da página -->
<link href="estilo.css" rel="stylesheet" type="text/css" />
<!-- Titulo da [ágina -->
<title><?php echo $titulo.' | '.ucwords($pagina) ?></title>
</head>
<body>
<div id="container"> <!--Conteudo do site!-->
<!-- Siga-nos.: <a target="_blank" href="#">
<img src ="<?php echo $facebook ?>" alt="<?php echo $empresa ?>" width="15" height="15" border="0" /></a>
<div id="alex"><NAME></div> -->
<div id="header"> <!--Primeira header da pagina!-->
<img id="logoImg" src="<?php echo $logoVM ?>" />
<div id="cabecalho">
<nav class="men">
<ul class="menu">
<li><a href="index.php">Home</a></li>
<li><a href="#produtos">Produtos</a></li>
<li><a href="#servicos">Serviços</a></li>
<li><a href="<?php echo '?p=empresa' ?>">Empresa</a></li>
<li><a href="faleconosco.php">Contato</a></li>
</ul>
</nav>
</div>
</div> <!--header-->
<file_sep>/footer.php
<!DOCTYPE html>
<html lang="pt-br">
<div class="clear"></div>
</div><!-- corpo -->
<div id="footer"> <!--Rodapé do site!-->
<?php echo $rodape ?>
</div><!--footer-->
</div><!-- container -->
</body>
</html>
| 869ee8229c5b6584ec37158ac52f7f11e012548e | [
"JavaScript",
"PHP"
] | 7 | PHP | alexandrego/VM | 6ca5b5ed9a71889dd5a6b800b1a5dca16391adc4 | a8d9213b30b77d367f257f79c44a05ca30b96b3e |
refs/heads/master | <file_sep>require 'test_helper'
class PostMastersHelperTest < ActionView::TestCase
end
<file_sep>class PostMastersController < ApplicationController
before_action :set_post_master, only: [:show, :edit, :update, :destroy]
# GET /post_masters
# GET /post_masters.json
def index
session[:post_id] = nil
@blog_master = BlogMaster.find(session[:blog_id])
@post_masters = PostMaster.where(blog_master_id: session[:blog_id])
end
# GET /post_masters/1
# GET /post_masters/1.json
def show
#@bolg_master = BlogMaster.where(blog_master_id: session[:blog_id])
@comment= Comment.where(post_master_id: @post_master.id)
#@comment.each do |c|
# @user = User.find(c.user_id)
# end
session[:post_id] = @post_master.id
end
# GET /post_masters/new
def new
@blog_master ||= BlogMaster.find(session[:blog_id])
@post_master = PostMaster.new
end
# GET /post_masters/1/edit
def edit
end
# POST /post_masters
# POST /post_masters.json
def create
@post_master = PostMaster.new(post_master_params)
respond_to do |format|
if @post_master.save
format.html { redirect_to @post_master, notice: 'Post master was successfully created.' }
format.json { render action: 'show', status: :created, location: @post_master }
else
format.html { render action: 'new' }
format.json { render json: @post_master.errors, status: :unprocessable_entity }
end
end
end
# PATCH/PUT /post_masters/1
# PATCH/PUT /post_masters/1.json
def update
respond_to do |format|
if @post_master.update(post_master_params)
format.html { redirect_to @post_master, notice: 'Post master was successfully updated.' }
format.json { head :no_content }
else
format.html { render action: 'edit' }
format.json { render json: @post_master.errors, status: :unprocessable_entity }
end
end
end
# DELETE /post_masters/1
# DELETE /post_masters/1.json
def destroy
@post_master.destroy
respond_to do |format|
format.html { redirect_to post_masters_url }
format.json { head :no_content }
end
end
private
# Use callbacks to share common setup or constraints between actions.
def set_post_master
@post_master = PostMaster.find(params[:id])
end
# Never trust parameters from the scary internet, only allow the white list through.
def post_master_params
params.require(:post_master).permit(:post_name, :discription, :blog_master_id)
end
end
<file_sep>require 'test_helper'
class BlogMastersControllerTest < ActionController::TestCase
setup do
@blog_master = blog_masters(:one)
end
test "should get index" do
get :index
assert_response :success
assert_not_nil assigns(:blog_masters)
end
test "should get new" do
get :new
assert_response :success
end
test "should create blog_master" do
assert_difference('BlogMaster.count') do
post :create, blog_master: { blog_name: @blog_master.blog_name, discription: @blog_master.discription, user_id: @blog_master.user_id }
end
assert_redirected_to blog_master_path(assigns(:blog_master))
end
test "should show blog_master" do
get :show, id: @blog_master
assert_response :success
end
test "should get edit" do
get :edit, id: @blog_master
assert_response :success
end
test "should update blog_master" do
patch :update, id: @blog_master, blog_master: { blog_name: @blog_master.blog_name, discription: @blog_master.discription, user_id: @blog_master.user_id }
assert_redirected_to blog_master_path(assigns(:blog_master))
end
test "should destroy blog_master" do
assert_difference('BlogMaster.count', -1) do
delete :destroy, id: @blog_master
end
assert_redirected_to blog_masters_path
end
end
<file_sep>/*
AiwinSlider created by <NAME>
Date created: 8-5-2014
Website: www.aiwin888.com
Version: 1
Description: The purpose of this plugin is to show the images from left to right and if it is
greater than the total images from the DOM. This works in IE 7 and above.
*/
(function ($) {
$(document).ready(function () {
$.fn.aiwinSlider = function (options) {
var $this = $(this);
var listImg = $(this).find("ul");
var listCont = $(this).find("li");
var countList = $(this).find("li").length;
var settings = $.extend({
nextPrevious: true,
paging: true,
animationInterval: 3000
}, options);
var containerWidth = listCont.width();
var assist = 0;
var intLine = 1;
$("#paging div:nth-child(0)").addClass("active");
setInterval(function () {
if (intLine == countList) {
listImg.animate({
"margin-left": "0px"
});
intLine = 1;
} else {
listImg.animate({
"margin-left": "-" + intLine++ * containerWidth
});
}
$("#paging div").removeClass("active");
$("#paging div:nth-child(" + intLine + ")").addClass("active");
}, settings.animationInterval);
$this.append("<div class='next'><img src='assets/right.png'></div>");
$this.append("<div class='prev'><img src='assets/left.png'></div>");
if (settings.nextPrevious) {
$(".next, .prev").show();
} else {
$(".next, .prev").hide();
}
$(".next").css("position", "absolute");
$(".next").css("top", "100px");
$(".next").css("right", "10px");
$(".prev").css("position", "absolute");
$(".prev").css("top", "100px");
$(".prev").css("left", "10px");
$(".next, .prev").css("cursor", "pointer");
$(".next").click(function () {
if (intLine == countList) {
listImg.animate({
"margin-left": "0px"
});
intLine = 1;
} else {
listImg.animate({
"margin-left": "-" + intLine++ * containerWidth
});
}
});
$(".prev").click(function () {
listImg.animate({
"margin-left": "-" + (--intLine) * containerWidth
});
});
$this.append("<div id='paging'></div>");
if (settings.paging) {
$("#paging").show();
} else {
$("#paging").hide();
}
$("#paging").css("position", "absolute");
for (var i = 0; i < countList; i++) {
$("#paging").append("<div></div>");
}
var pageNum = $("#paging div");
pageNum.click(function () {
var index = pageNum.index(this);
listImg.animate({
"margin-left": "-" + index * containerWidth
});
intLine = index;
$("#paging div").removeClass("active");
$("#paging div:nth-child(" + (index + 1) + ")").addClass("active");
});
};
});
} (jQuery));<file_sep>class CreatePostMasters < ActiveRecord::Migration
def change
create_table :post_masters do |t|
t.string :post_name
t.string :discription
t.integer :blog_master_id
t.timestamps
end
end
end
<file_sep>module PostMastersHelper
def bg_select(post_count)
if post_count == 3
style = "background-color: #81BEF7"
else
style = "background-color: white"
end
end
end
<file_sep>class PostMaster < ActiveRecord::Base
belongs_to :blog_master
has_many :comment
validates :post_name, :discription, presence: true
end
<file_sep>require 'spec_helper'
describe PostMastersController do
end
<file_sep>require 'test_helper'
class BlogMastersHelperTest < ActionView::TestCase
end
<file_sep>class User < ActiveRecord::Base
# Include default devise modules. Others available are:
# :confirmable, :lockable, :timeoutable and :omniauthable
has_many :blog_master
has_many :comment
devise :database_authenticatable, :registerable,
:recoverable, :rememberable, :trackable, :validatable
after_create :welcome_message
private
def welcome_message
#UserMailer.welcome_message(self).deliver
Rails.logger.info("@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@");
UserNotifier.send_signup_email(self).deliver
end
end
<file_sep># namespace :db do
# desc "Truncate all tables"
# task :truncate => :environment do
# conn = ActiveRecord::Base.connection
# tables = conn.execute("show tables").map { |r| r[0] }
# tables.delete "schema_migrations"
# tables.each { |t| conn.execute("DELETE FROM #{t}") }
# # connection = ActiveRecord::Base.connection
# # connection.disable_referential_integrity do
# # connection.tables.each do |table_name|
# # next if connection.select_value("SELECT count(*) FROM #{table_name}") == 0
# # connection.execute("DELETE FROM #{table_name}")
# # end
# end
# end
namespace :db do
desc "Truncate all existing data"
task :truncate => "db:load_config" do
begin
config = ActiveRecord::Base.configurations[::Rails.env]
ActiveRecord::Base.establish_connection
case config["adapter"]
when "mysql", "postgresql"
ActiveRecord::Base.connection.tables.each do |table|
ActiveRecord::Base.connection.execute("TRUNCATE #{table}")
end
when "sqlite", "sqlite3"
ActiveRecord::Base.connection.tables.each do |table|
ActiveRecord::Base.connection.execute("DELETE FROM #{table}")
ActiveRecord::Base.connection.execute("DELETE FROM sqlite_sequence where name='#{table}'")
end
ActiveRecord::Base.connection.execute("VACUUM")
end
end
end
end<file_sep>class Comment < ActiveRecord::Base
belongs_to :post_master
belongs_to :user
end
<file_sep>class BlogMaster < ActiveRecord::Base
belongs_to :user
has_many :post_master
validates :blog_name,:discription, presence: true
end
<file_sep>
class BlogMastersController < ApplicationController
before_action :set_blog_master, only: [:show, :edit, :update, :destroy]
# GET /blog_masters
# GET /blog_masters.json
def index
#list_of_column(blog_master)
session[:blog_id] = nil
@blog_masters = BlogMaster.where(user_id: current_user)
@admin_blog = BlogMaster.find(:all)
@admin_user = User.where(role: 'user')
end
# GET /blog_masters/1
# GET /blog_masters/1.json
def show
session[:blog_id] = @blog_master.id
redirect_to post_masters_path
end
# GET /blog_masters/new
def new
@blog_master = BlogMaster.new
end
# GET /blog_masters/1/edit
def edit
end
# POST /blog_masters
# POST /blog_masters.json
def create
@blog_master = BlogMaster.new(blog_master_params)
respond_to do |format|
if @blog_master.save
format.html { redirect_to blog_masters_path, notice: 'Blog master was successfully created.' }
format.json { render action: 'show', status: :created, location: @blog_master }
else
format.html { render action: 'new' }
format.json { render json: @blog_master.errors, status: :unprocessable_entity }
end
end
end
# PATCH/PUT /blog_masters/1
# PATCH/PUT /blog_masters/1.json
def update
respond_to do |format|
if @blog_master.update(blog_master_params)
format.html { redirect_to blog_masters_path, notice: 'Blog master was successfully updated.' }
format.json { head :no_content }
else
format.html { render action: 'edit' }
format.json { render json: @blog_master.errors, status: :unprocessable_entity }
end
end
end
# DELETE /blog_masters/1
# DELETE /blog_masters/1.json
def destroy
@blog_master.destroy
respond_to do |format|
format.html { redirect_to blog_masters_url }
format.json { head :no_content }
end
end
private
# Use callbacks to share common setup or constraints between actions.
def set_blog_master
@blog_master = BlogMaster.find(params[:id])
end
# Never trust parameters from the scary internet, only allow the white list through.
def blog_master_params
params.require(:blog_master).permit(:blog_name, :discription, :user_id)
end
end
<file_sep>json.array!(@post_masters) do |post_master|
json.extract! post_master, :post_name, :discription, :blog_master_id
json.url post_master_url(post_master, format: :json)
end
<file_sep>json.array!(@blog_masters) do |blog_master|
json.extract! blog_master, :blog_name, :discription, :user_id
json.url blog_master_url(blog_master, format: :json)
end
<file_sep>require 'spec_helper'
describe BlogMastersController do
end
<file_sep>json.extract! @post_master, :post_name, :discription, :blog_master_id, :created_at, :updated_at
<file_sep>require 'test_helper'
class PostMastersControllerTest < ActionController::TestCase
setup do
@post_master = post_masters(:one)
end
test "should get index" do
get :index
assert_response :success
assert_not_nil assigns(:post_masters)
end
test "should get new" do
get :new
assert_response :success
end
test "should create post_master" do
assert_difference('PostMaster.count') do
post :create, post_master: { blog_master_id: @post_master.blog_master_id, discription: @post_master.discription, post_name: @post_master.post_name }
end
assert_redirected_to post_master_path(assigns(:post_master))
end
test "should show post_master" do
get :show, id: @post_master
assert_response :success
end
test "should get edit" do
get :edit, id: @post_master
assert_response :success
end
test "should update post_master" do
patch :update, id: @post_master, post_master: { blog_master_id: @post_master.blog_master_id, discription: @post_master.discription, post_name: @post_master.post_name }
assert_redirected_to post_master_path(assigns(:post_master))
end
test "should destroy post_master" do
assert_difference('PostMaster.count', -1) do
delete :destroy, id: @post_master
end
assert_redirected_to post_masters_path
end
end
<file_sep>json.extract! @blog_master, :blog_name, :discription, :user_id, :created_at, :updated_at
| c84e33c8c51760bb70cca743cd3a8dd73e71e643 | [
"JavaScript",
"Ruby"
] | 20 | Ruby | twinks14/blog | 1fccae9bd3f364cc036a28624b99887adad7151d | eaeaf1dc76ed4d6fd6933863fa8ec13c0e2c7f35 |
refs/heads/master | <repo_name>EmmanuelCruz/Complejidad_Computacional<file_sep>/README.md
# Complejidad Computacional
## <NAME>
### Programas
1. Implementación del problema de _alcanzabilidad_ y _conjunto dominante_.
2. Corrección de la implementación de _alcanzabilidad_ y _conjunto dominante_.
3. Implementación de _SubsetSum_ y _TSP_.
4. Solución al problema de las _N reinas_ con _Algoritmos genéticos_ y _Recocido Simulado_.
### Tareas
Tareas de investigación del curso de Complejidad Computacional.
<file_sep>/Programas/Programa01/Dominante/src/fciencias/complejidad/programa1/Dominante.java
package fciencias.complejidad.programa1;
import java.util.ArrayList;
import java.util.Random;
import java.util.Scanner;
/**
* Implementación para calcular un conjunto dominante de una gráfica.
* @author <NAME>,
* @version 1.0 Octubre 2020.
* @since Complejidad Computacional 2021-1.
*/
public class Dominante{
/**
* Clase que representa un par.
*/
private class Pair<T, U>{
/** Llave del par. */
private T llave;
/** Valor asociado. */
private U valor;
public Pair(T t, U u){
this.llave = t;
this.valor = u;
}
public T getKey(){
return llave;
}
public U getValue(){
return valor;
}
}
/** Grafica a buscar alcanzabilidad entre <i>s</i> y <i>t</i>. */
private Grafica g;
/**
* Crea un nuevo dominante.
* @param n la cantidad de vértices.
*/
public Dominante(int n){
Random rn = new Random();
g = new Grafica(n);
int aristas = n*(n-1)/2;
for(int i = 0; i<aristas; i++){
int a = rn.nextInt(n);
int b = rn.nextInt(n);
g.insertaArista(a, b);
}
}
/**
* Crea un nuevo dominante con una gráfica específica.
* @param g la gráfica donde buscar calcular conjunto dominante.
*/
public Dominante(Grafica g){
this.g = g;
}
/**
* Implementación de la búsqueda del conjunto dominante.
* @return una lista con los identificadores de los vértices del conjunto dominante
*/
public ArrayList<Integer> conjuntoDominante(){
ArrayList<Pair<Integer, Integer>> ordenada = mergeSortModificado(this.generaGrados());
ArrayList<Integer> dominante = new ArrayList<>();
ArrayList<Integer> eliminados = new ArrayList<>();
for(int i = 0; i<g.longitud(); i++)
dominante.add(i);
for(int i = 0; i<ordenada.size(); i++){
int id = ordenada.get(i).getKey();
int pos = dominante.indexOf(id);
int eliminado = dominante.remove(pos);
boolean adyacente = false;
for(int j = 0; j<dominante.size(); j++)
if(g.esAdyacente(eliminado, dominante.get(j))){
adyacente = true;
break;
}
if(!adyacente)
dominante.add(eliminado);
for(int w : eliminados){
adyacente = false;
for(int v : dominante)
if(g.esAdyacente(w, v)){
adyacente = true;
break;
}
if(!adyacente){
dominante.add(eliminado);
break;
}
}
if(adyacente)
eliminados.add(eliminado);
}
return dominante;
}
/**
* Regresa una lista de vértices con sus grados.
* @return la lista de vértices con su grados.
*/
public ArrayList<Pair<Integer, Integer>> generaGrados(){
ArrayList<Pair<Integer, Integer>> vertices = new ArrayList<>();
for(int i = 0; i<g.longitud(); i++){
Pair<Integer, Integer> nuevo = new Pair<>(i, g.daGrado(i));
vertices.add(nuevo);
}
return vertices;
}
/**
* Merge sort modificado para ordenar el valor de pares con su llave.
* @param lista la lista de pares a ordenar.
* @return la lista de pares ordenada.
*/
private ArrayList<Pair<Integer, Integer>> mergeSortModificado(ArrayList<Pair<Integer, Integer>> lista){
if(lista.size()==1)
return lista;
int mitad = lista.size()/2;
ArrayList<Pair<Integer, Integer>> l1 = sublista(0, mitad, lista);
ArrayList<Pair<Integer, Integer>> l2 = sublista(mitad, lista.size(), lista);
l1 = mergeSortModificado(l1);
l2 = mergeSortModificado(l2);
return mergeAux(l1, l2);
}
/**
* Corta una lista en un rango de la lista.
* @param i el inicio de corte.
* @param f el fin de corte.
* @param lista la lista a subdividir.
* @return una sublista desde el índice i al índice f.
*/
private ArrayList<Pair<Integer, Integer>> sublista(int i, int f,
ArrayList<Pair<Integer, Integer>> lista){
ArrayList<Pair<Integer, Integer>> sublista = new ArrayList<>();
for(int j = i; j<f ; j++)
sublista.add(lista.get(j));
return sublista;
}
/**
* Auxiliar de merge sort modificado para ordenar los elementos.
* @param l1 la primera lista a ordenar elementos.
* @param l2 la segunda lista a ordenar elementos.
* @param una lista ordenada a partir de las listas l1 y l2.
*/
private ArrayList<Pair<Integer, Integer>> mergeAux(ArrayList<Pair<Integer, Integer>> l1,
ArrayList<Pair<Integer, Integer>> l2){
ArrayList<Pair<Integer, Integer>> ordenada = new ArrayList<>();
while(!l1.isEmpty() && !l2.isEmpty())
if(l1.get(0).getValue() < l2.get(0).getValue())
ordenada.add(l1.remove(0));
else
ordenada.add(l2.remove(0));
ordenada.addAll(l1);
ordenada.addAll(l2);
return ordenada;
}
/**
* Verifica si un conjunto es conjunto dominante.
* @param conjunto una lista de vértices candidatos a ser conjunto
* dominante.
* @return true si el conjunto es conjunto dominante, false en otro caso.
*/
public boolean esDominante(ArrayList<Integer> conjunto){
ArrayList<Integer> complemento = new ArrayList<>();
if(conjunto.isEmpty())
return false;
for(int i = 0; i<g.longitud(); i++)
complemento.add(i);
complemento.removeAll(conjunto);
for(int i : complemento)
for(int w : conjunto)
if(!g.esAdyacente(i, w))
return false;
return true;
}
/**
* Imprime los elementos de una lista en forma de conjunto.
* @param lista la lista a representar elementos.
* @return una representación en conjunto de los elementos de una lista.
*/
public static String muestra(ArrayList<Integer> lista){
String conjunto = "| ";
for(int w : lista)
conjunto += w+" | ";
return conjunto;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Dominante dominante = null;
/* Colores */
String red = "\033[31m";
String green = "\033[32m";
String yellow = "\033[33m";
String blue = "\033[34m";
String cyan = "\033[36m";
String reset = "\u001B[0m";
int opcion = Integer.parseInt(args[0]);
int vertices = 0;
if(opcion == 1){
System.out.println(cyan+"\nHas elegido crear una nueva gráfica");
System.out.println(reset+"\nIngresa la cantidad de vértices que deseas en la gráfica");
vertices = sc.nextInt();
Grafica nueva = new Grafica(vertices);
System.out.println(reset+"\nIngresa las aristas de la gráfica con el formato: a b"+blue+
"\nPor ejemplo, si quiero hacer una arista entre el vértice "+
"0 y el vértice 5,\nen la línea pondría: 0 5"+reset+
"\nPara terminar la ejecución ingresa: -1 -1\n");
int a = 0;
int b = 0;
while(true){
try{
a = sc.nextInt();
b = sc.nextInt();
if(a==-1 && b==-1)
break;
nueva.insertaArista(a, b);
} catch(IllegalArgumentException iae){
System.out.println(red + "\nIngresaste un identificador fuera de rango" + reset);
} catch(Exception e){
System.out.println(red + "\nIngresaste un identificador inválido" + reset);
sc.nextLine();
}
}
dominante = new Dominante(nueva);
} else if(opcion == 0){
System.out.println(cyan+"\nHas elegido usar la gráfica generada aleatoriamente");
System.out.println(reset+"\nIngresa la cantidad de vértices que deseas en la gráfica");
vertices = sc.nextInt();
dominante = new Dominante(vertices);
} else
return;
System.out.println(green+"La gráfica de entrada está representada por la matriz de adyacencia:\n"+reset+dominante.g);
System.out.println(reset+"\nIngresa los vértices del conjunto a verificar si es conjunto dominante"+blue+
"\nCada uno de los vértices debe ser un identifiador de tipo entero entre 0 y "+(dominante.g.longitud()-1)+
"\nPara terminar la ejecución ingresa: -1\n"+reset);
ArrayList<Integer> entrada = new ArrayList<>();
int vert = -2;
while(vert != -1){
try{
vert = sc.nextInt();
if(vert == -1)
break;
if(vert < 0 || vert>=dominante.g.longitud())
continue;
entrada.add(vert);
} catch(Exception e){
System.out.println(red + "\nIngresaste un identificador inválido" + reset);
sc.nextLine();
}
}
System.out.println(reset+"¿El conjunto "+green+(muestra(entrada))+reset+" es dominante? "+
(dominante.esDominante(entrada)?(green+"Si es dominante!"):(red+"No es dominante :("))+reset);
ArrayList<Integer> resultado = dominante.conjuntoDominante();
String conjunto = muestra(resultado);
System.out.println(green+"\nUn conjunto dominante es: "+conjunto);
}
}<file_sep>/Programas/Programa03/README.md
# Problema de las N Reinas.
## Autor: <NAME>.
Se encuentran dos directorios:
### Algoritmos Genéticos
Para compilar el programa se usa el comando:
javac ReinasGenetico.java
Para correr el programa se usa el comando:
java ReinasGenetico
### Recocido Simulado
Para compilar el programa se usa el comando:
javac ReinasRecocido.java
Para correr el programa se usa el comando:
java ReinasRecocido<file_sep>/Programas/Programa02/README.md
# Algoritmos de apoximación. TSP-Metric y Subset Sum.
## <NAME>.
### Subset Sum
Para compilar y ejecutar la implementación del algoritmo se utilizan los siguientes comandos dentro del directorio llamado SubsetSum.
1. javac SubsetSum.java
Para compilar el código.
2. java SubsetSum
Para ejecutar el código.
Se solicita que el usuario ingrese los valores con los cuales va a trabajar el algoritmo. Primero se solicita la cantidad de pesos a considerar, después los pesos, seguido de un entero t que define el límite de pesos y finalmente un número de punto flotante entre 0 y 1. Se muestran tres listas en cada iteración, la primera corresponde a merge, la segunda corresponde a trim y la última corresponde a la lista sin los elementos mayores a t.
### TSP-Metric.
Para poder compilar y ejecutar la implementación se utilizan los siguientes comandos en una terminal dentro del directorio llamado TSP:
1. javac Arista.java Grafica.java TSPMetric.java
Para compilar las clases.
2. java TSPMetric
Para correr el programa.
NOTA: Se anexa un PDF con el proceso que sigue el programa para encontrar la solución con una gráfica ya definida en el programa. Las aristas se encuentran definidas en el método construyeEjemplar() en la clase TSPMetric.<file_sep>/Programas/Programa01/Dominante/README.md
# Conjunto Dominante
## Autor: <NAME>.
Para ejecutar el proyecto se usan los siguientes comandos:
### ant build
Para construir los directorios donde se guardarán los ejecutables y todo lo necesario para el programa.
### ant jar
Para construir los archivos .class en carpetas .jar que nos permitirá ejecutar el programa.
### ant -Darg0=0 run
Permite correr el programa usando una gráfica generada aleatoriamente.
### ant -Darg0=1 run
Permite correr el programa usando una gráfica creada por el usuario a partir de n cantidad de aristas y agregar los vértices de la gráfica por pares de identificadores.
### ant clean
Permite limpiar todas los directorios creados sobre el programa. Es muy recomendable después de usar el programa.<file_sep>/Programas/Programa01 (Reposición)/Dominante/README.md
# Conjutno Dominante
## Autor: <NAME>.
Para ejecutar el proyecto se usan los siguientes comandos, en una ventana de la terminal posicionada en el directorio src:
### javac *.java
Para compilar el código en Java.
### java Dominante
Permite correr el programa, mostrando los resultado en terminal.
<file_sep>/Programas/Programa01/Alcanzabilidad/src/fciencias/complejidad/programa1/Alcanzable.java
package fciencias.complejidad.programa1;
import java.lang.IllegalArgumentException;
import java.util.ArrayList;
import java.util.ArrayDeque;
import java.util.Scanner;
import java.util.Random;
/**
* Se implementa el problema de decisión <i>Alcanzabilidad</i>.
* @author <NAME>.
* @version 1.0 Octubre 2020.
* @since Complejidad Computacional 2021-1.
*/
public class Alcanzable{
/** Grafica a buscar alcanzabilidad entre <i>s</i> y <i>t</i>. */
private Grafica g;
/**
* Crea un nuevo Alcanzable.
* @param n la cantidad de vértices.
*/
public Alcanzable(int n){
Random rn = new Random();
g = new Grafica(n);
int aristas = n*(n-1)/2;
for(int i = 0; i<aristas; i++){
int a = rn.nextInt(n);
int b = rn.nextInt(n);
g.insertaArista(a, b);
}
}
/**
* Crea un nuevo alcanzable con una gráfica específica.
* @param g la gráfica donde buscar alcanzabilidad.
*/
public Alcanzable(Grafica g){
this.g = g;
}
/**
* BFS - Breadth-First Search. Búsqueda en anchura sobre una
* gráfica implementada con una matriz de adyacencias.
* @param v el vértice de inicio.
* @return la lista de todos los vértices alcanzados desde
* un vértice inicial.
* @throws IllegalArgumentException si el identificador es inválido.
*/
public ArrayList<Integer> applyBFS(int v){
if(v<0 || v>=g.longitud())
throw new IllegalArgumentException("El vérice no es válido");
ArrayDeque<Integer> pendientes = new ArrayDeque<>();
ArrayList<Integer> visitados = new ArrayList<>();
visitados.add(v);
pendientes.add(v);
while(!pendientes.isEmpty()){
int u = pendientes.remove();
ArrayList<Integer> vecinosU = g.daVecinos(u);
for(int w : vecinosU)
if(!visitados.contains(w)){
visitados.add(w);
pendientes.add(w);
}
}
return visitados;
}
/**
* Verifica si un vértice <i>t</i> es alcanzable desde
* un vértice <i>s</i>.
* @param s el vértice inicial a buscar alcanzabilidad.
* @param t el vértice a verificar alcanzabilidad desde <i>i</i>.
* @return true si los vértices son alcanzables, false en otro caso.
* @throws IllegalArgumentException si alguno de los identificadores son inválidos.
*/
public boolean esAlcanzable(int s, int t) throws IllegalArgumentException{
if(s<0 || s>=g.longitud())
throw new IllegalArgumentException("El vérice s no es válido");
if(t<0 || t>=g.longitud())
throw new IllegalArgumentException("El segundo vértice no es válido");
ArrayList<Integer> alcanzados = this.applyBFS(s);
return alcanzados.contains(t);
}
/**
* Encuentra un camino entre dos vértices.
* @param v1 el vértice de inicio.
* @param v2 el vértice fin.
* @return un camino entre ambos vértices.
*/
public ArrayList<Integer> encuentraCamino(int v1, int v2){
ArrayList<Integer> camino = new ArrayList<>();
camino.add(v1);
ArrayList<Integer> visitados = new ArrayList<>();
visitados.add(v1);
return encuentraCamino(v2, camino, visitados);
}
/**
* Encuentra un camino al vértice v
* @param vértice a buscar.
*/
private ArrayList<Integer> encuentraCamino(int v, ArrayList<Integer> camino,
ArrayList<Integer> visitados){
int ultimo = camino.get(camino.size()-1);
if(ultimo == v)
return camino;
ArrayList<Integer> vecinos = g.daVecinos(ultimo);
vecinos.remove(visitados);
if(vecinos.isEmpty()){
camino.remove(ultimo);
return camino;
}
for(int w : vecinos){
ArrayList<Integer> caminoAux = new ArrayList<>();
caminoAux.addAll(0, camino);
caminoAux.add(w);
ArrayList<Integer> visitadosAux = new ArrayList<>();
visitadosAux.addAll(0, visitados);
if(!visitadosAux.contains(w)){
visitadosAux.add(w);
ArrayList<Integer> posible = encuentraCamino(v, caminoAux, visitadosAux);
if(posible.get(posible.size()-1) == v)
return posible;
}
}
return new ArrayList<Integer>();
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Alcanzable alcanzable = null;
/* Colores */
String red = "\033[31m";
String green = "\033[32m";
String yellow = "\033[33m";
String blue = "\033[34m";
String cyan = "\033[36m";
String reset = "\u001B[0m";
int s = 0;
int t = 0;
int opcion = Integer.parseInt(args[0]);
int vertices = 0;
if(opcion == 1){
System.out.println(cyan+"\nHas elegido crear una nueva gráfica");
System.out.println(reset+"\nIngresa la cantidad de vértices que deseas en la gráfica");
vertices = sc.nextInt();
Grafica nueva = new Grafica(vertices);
System.out.println(reset+"\nIngresa las aristas de la gráfica con el formato: a b"+blue+
"\nPor ejemplo, si quiero hacer una arista entre el vértice "+
"0 y el vértice 5,\nen la línea pondría: 0 5"+reset+
"\nPara terminar la ejecución ingresa: -1 -1\n");
int a = 0;
int b = 0;
while(true){
try{
a = sc.nextInt();
b = sc.nextInt();
if(a==-1 && b==-1)
break;
nueva.insertaArista(a, b);
} catch(IllegalArgumentException iae){
System.out.println(red + "\nIngresaste un identificador fuera de rango" + reset);
} catch(Exception e){
System.out.println(red + "\nIngresaste un identificador inválido" + reset);
sc.nextLine();
}
}
alcanzable = new Alcanzable(nueva);
} else if(opcion == 0){
System.out.println(cyan+"\nHas elegido usar la gráfica generada aleatoriamente");
System.out.println(reset+"\nIngresa la cantidad de vértices que deseas en la gráfica");
vertices = sc.nextInt();
alcanzable = new Alcanzable(vertices);
} else
return;
System.out.println(green+"La gráfica de entrada está representada por la matriz de adyacencia:\n"+reset+alcanzable.g);
while(true){
System.out.println(reset+"\nPara salir ingresa como indentificadores: -1 -1");
System.out.println(reset+"\nIngresa el identificador del vértice "+yellow+
"s"+reset+" ([0, "+(alcanzable.g.longitud()-1)+"])");
try{
s = sc.nextInt();
} catch(Exception e){
System.out.println(red + "Ingresaste un identificador inválido");
sc.nextLine();
continue;
}
System.out.println(reset+
"\nIngresa el identificador del vértice "+yellow+
"t"+reset+" ([0, "+(alcanzable.g.longitud()-1)+"])");
try{
t = sc.nextInt();
} catch(Exception e){
System.out.println(red + "Ingresaste un identificador inválido");
sc.nextLine();
continue;
}
if(s==-1 && t==-1){
System.out.println(yellow + "\nSALIENDO . . .");
return;
}
try{
System.out.println(reset+"\n¿Es alcanzable el vértice "+t+" desde "+s+"?");
boolean esAlcanzable = alcanzable.esAlcanzable(s, t);
System.out.println("\n"+(esAlcanzable?green+"SI ES ALCANZABLE!":
red+"NO ES ALCANZABLE :(")+"\n");
if (esAlcanzable) {
ArrayList<Integer> camino = alcanzable.encuentraCamino(s, t);
String caminoCreado = "";
for(int ver : camino)
caminoCreado = "-> "+ver;
System.out.println("Uno de lso caminos posibles es: "+camino);
}
} catch(IllegalArgumentException iae){
System.out.println(red + "Ingresaste un identificador fuera de rango");
}
}
}
}<file_sep>/Programas/Programa01 (Reposición)/Alcanzabilidad/src/Alcanzable.java
import java.lang.IllegalArgumentException;
import java.util.ArrayList;
import java.util.ArrayDeque;
import java.util.Scanner;
import java.util.Random;
/**
* Se implementa el problema de decision <i>Alcanzabilidad</i>.
* @author <NAME>.
* @version 2.0 Enero 2021 (Antigua version 1.0 Octubre 2020).
* @since Complejidad Computacional 2021-1.
*/
public class Alcanzable{
/** Grafica a buscar alcanzabilidad entre <i>s</i> y <i>t</i>. */
private Grafica g;
/**
* Crea un nuevo Alcanzable.
* @param n la cantidad de vertices.
*/
public Alcanzable(){
}
/**
* Crea una grafica de forma aleatoria.
* @param n la cantidad de vertices de la grafica.
*/
public void creaGrafica(int n){
Random rn = new Random();
g = new Grafica(n);
for(int i = 0; i<n; i++)
for(int j = i+1 ; j<n ; j++){
boolean moneda = rn.nextBoolean();
if(moneda)
g.insertaArista(i, j);
}
}
/**
* BFS - Breadth-First Search. Busqueda en anchura sobre una
* grafica implementada con una matriz de adyacencias.
* @param v el vertice de inicio.
* @return la lista de todos los vertices alcanzados desde
* un vertice inicial.
* @throws IllegalArgumentException si el identificador es invalido.
*/
public ArrayList<Integer> applyBFS(int v){
if(v<0 || v>=g.longitud())
throw new IllegalArgumentException("El verice no es valido");
ArrayDeque<Integer> pendientes = new ArrayDeque<>();
ArrayList<Integer> visitados = new ArrayList<>();
visitados.add(v);
pendientes.add(v);
while(!pendientes.isEmpty()){
int u = pendientes.remove();
ArrayList<Integer> vecinosU = g.daVecinos(u);
for(int w : vecinosU)
if(!visitados.contains(w)){
visitados.add(w);
pendientes.add(w);
}
}
return visitados;
}
/**
* Verifica si un vertice <i>t</i> es alcanzable desde
* un vertice <i>s</i>.
* @param s el vertice inicial a buscar alcanzabilidad.
* @param t el vertice a verificar alcanzabilidad desde <i>i</i>.
* @return true si los vertices son alcanzables, false en otro caso.
* @throws IllegalArgumentException si alguno de los identificadores son invalidos.
*/
public boolean esAlcanzable(int s, int t) throws IllegalArgumentException{
if(s<0 || s>=g.longitud())
throw new IllegalArgumentException("El verice s no es valido");
if(t<0 || t>=g.longitud())
throw new IllegalArgumentException("El segundo vertice no es valido");
ArrayList<Integer> alcanzados = this.applyBFS(s);
return alcanzados.contains(t);
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Alcanzable alcanzable = new Alcanzable();
int s = 0;
int t = 0;
System.out.println("\nIngresa la cantidad de vertices que deseas en la grafica");
int vertices = sc.nextInt();
alcanzable.creaGrafica(vertices);
System.out.println("La grafica generada aleatoriamente por la fase adivinadora es:\n"+alcanzable.g);
while(true){
System.out.println("\nPara salir ingresa como indentificadores: -1 -1");
System.out.println("\nIngresa el identificador del vertice s ([0, "+(alcanzable.g.longitud()-1)+"])");
try{
s = sc.nextInt();
} catch(Exception e){
System.out.println("Ingresaste un identificador invalido");
sc.nextLine();
continue;
}
System.out.println("\nIngresa el identificador del vertice t ([0, "+(alcanzable.g.longitud()-1)+"])");
try{
t = sc.nextInt();
} catch(Exception e){
System.out.println("Ingresaste un identificador invalido");
sc.nextLine();
continue;
}
if(s==-1 && t==-1){
System.out.println("\nSALIENDO . . .");
return;
}
try{
System.out.println("\n¿Es alcanzable el vertice "+t+" desde "+s+"?");
boolean esAlcanzable = alcanzable.esAlcanzable(s, t);
System.out.println("\n"+(esAlcanzable?"SI ES ALCANZABLE!":
"NO ES ALCANZABLE :(")+"\n");
} catch(IllegalArgumentException iae){
System.out.println("Ingresaste un identificador fuera de rango");
}
}
}
}<file_sep>/Programas/Programa03/Recocido Simulado/ReinasRecocido.java
import java.util.Random;
import java.util.Scanner;
/**
* Clase en la que se implementa el uso de recodido simulado
* para resolver el problema de las n reinas.
* @author <NAME>. 314272588.
* @version 0.1 Enero 2021.
*/
public class ReinasRecocido{
/**
* Clase auxiliar que representa un Reina de reinas.
*/
public class Reina{
/** Representación de un Reina de nxn. */
public int[] tablero;
/** Costo del tablero. */
public double costo;
private Random rnd=new Random();
/** Tabla de colisiones. */
public boolean[] colisiones;
/**
* Crea un nuevo Reina.
* @param n el tamaño del Reina para que se represente de nxn.
*/
public Reina(int n){
tablero=new int[n];
for(int i=0;i<n;i++){
tablero[i]=rnd.nextInt(n);
}
colisiones = new boolean[n];
this.calculacosto();
}
/**
* Crea un Reina a partir de un arreglo ya dado.
* @param tab el nuevo tablero a asignar al objeto.
*/
public Reina(int[] tab){
tablero=tab;
colisiones = new boolean[tab.length];
this.calculacosto();
}
/**
* Calcula la costo del Reina.
* @return la cantidad de ataques entre reinas en el Reina.
* NOTA: Si una reina r1 ya atacó a la reina r2 y se contabilizó entonces el ataque entre r2 y r1 no se cuenta.
*/
public double calculacosto(){
int contador=0;
int diagonal1=0;
int diagonal2=0;
int recta=0;
// Se reorre el individuo para detectar la cantidad de reinas que se comen entre sí.
for(int i=0;i<tablero.length;i++){
diagonal1=0;
diagonal2=0;
// Por cada entrada del individuo cuenta las veces que se comen una reina.
for(int j=i;j<tablero.length;j++){
// Caso para las reinas en el mismo renglón.
if(tablero[i]==tablero[j]){
colisiones[i] = true;
colisiones[j] = true;
contador++;
}
// Caso para las reinas en la misma diaonal \
if(tablero[i]+diagonal2 == tablero[j]){
colisiones[i] = true;
colisiones[j] = true;
contador++;
}
diagonal2++;
// Caso para las reinas en la misma diaonal /
if(tablero[i]+diagonal1 == tablero[j]){
colisiones[i] = true;
colisiones[j] = true;
contador++;
}
diagonal1--;
}
contador=contador-3;
}
// Actualizamos la costo.
costo=contador;
return contador;
}
@Override
public String toString(){
String representacion="[";
for(int i=0;i<tablero.length;i++)
representacion+=tablero[i]+" ";
representacion+="]\n\n";
for(int j=0;j<tablero.length;j++){
for(int k=0;k<tablero.length;k++){
if(tablero[j]==k)
representacion+="R ";
else
representacion+="* ";
}
representacion+="\n";
}
representacion+="costo = "+costo+".\n";
return representacion;
}
}
/**
* Implementación de recocido simulado para encontrar la mejor solución
* de las n reinas.
* @param tam la longitud del tablero de reinas.
*/
public Reina recocidoSimulado(int tam){
double t = 10;
double n = 1;
Random rn = new Random();
Reina ri = new Reina(tam);
while(ri.costo > 0 && t > 0){
int[] rjTab = new int[tam];
for(int i = 0; i < rjTab.length; i++){
if(ri.colisiones[i]){ // Se le da mas probabilidad a las colisiones
if(rn.nextDouble() < 0.8 )
rjTab[i] = rn.nextInt(tam);
} else if( rn.nextDouble() < 0.4){
rjTab[i] = rn.nextInt(tam);
} else{
rjTab[i] = ri.tablero[i];
}
}
Reina rj = new Reina(rjTab);
if(rj.costo <= ri.costo)
ri = rj;
else
if(Math.exp((ri.costo - rj.costo) / t) > rn.nextDouble())
ri = rj;
t *= 0.80 ;
n *= 2;
}
return ri;
}
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("Ingresa la cantidad de reinas a encontrar solución");
int cantidadReinas = sc.nextInt();
ReinasRecocido tn = new ReinasRecocido();
/*
System.out.println("Ingresa la cantidad de iteeraciones para crear generaciones");
int iteraciones = sc.nextInt();
*/
Reina solucionFinal=tn.recocidoSimulado(cantidadReinas);
System.out.println("MEJOR SOLUCIÓN ENCONTRADA\n"+solucionFinal);
}
}
| bb32028b76372ff095c02cf3883f31442e0a0465 | [
"Markdown",
"Java"
] | 9 | Markdown | EmmanuelCruz/Complejidad_Computacional | 188c6512abe91e391bf88e149a1ecdac0489698a | f285805903a22ebc100921ab70fe4471726b7791 |
refs/heads/main | <repo_name>Momentum-Team-9/react-router-example<file_sep>/src/components/BookForm.js
import { useState } from 'react'
import axios from 'axios'
export const BookForm = ({ token, setSubmitted }) => {
const [title, setTitle] = useState('')
const [author, setAuthor] = useState('')
const [pubDate, setPubDate] = useState('')
const handleSubmit = (event) => {
event.preventDefault()
axios.post('https://drf-library-api.herokuapp.com/api/books',
{
title: title,
author: author,
publication_date: pubDate
},
{
headers: {
'Content-Type': 'application/json',
Authorization: `token ${token}`
}
}
).then(res => {
setSubmitted(true)
setTitle('')
setAuthor('')
setPubDate('')
return res
})
}
const handleChange = (inputType, event) => {
if (inputType === 'title') {
setTitle(event.target.value)
}
if (inputType === 'author') {
setAuthor(event.target.value)
}
if (inputType === 'publication date') {
setPubDate(event.target.value)
}
}
return (
<div className='uk-container uk-flex uk-flex-center uk-flex-middle uk-height-large'>
<form className='uk-form-horizontal' onSubmit={handleSubmit}>
<label className='uk-form-label'>Title</label>
<input
className='uk-input'
type='text'
placeholder='Book title'
value={title}
onChange={(e) => handleChange('title', e)}
/>
<label className='uk-form-label'>Author</label>
<input
className='uk-input'
placeholder='Author'
type='text'
// using state to pass a value to this attribute
// makes this a controlled component
value={author}
onChange={(e) => handleChange('author', e)}
/>
<label className='uk-form-label'>Publication Date</label>
<input
className='uk-input uk-margin-bottom'
placeholder='Publication date'
type='text'
// using state to pass a value to this attribute
// makes this a controlled component
value={pubDate}
onChange={(e) => handleChange('publication date', e)}
/>
<button className='uk-button'>Submit</button>
</form>
</div>
)
}
<file_sep>/src/components/Navbar.js
import '../styles.css'
import { Link, useHistory } from 'react-router-dom'
export const Navbar = ({ token, setAuth, clearStorage }) => {
const history = useHistory()
return (
<nav className='uk-navbar uk-navbar-container' uk-navbar>
<div className='uk-navbar-left'>
<Link className='uk-logo uk-margin-left' to='/' onClick={() => history.push('/')}>Bookify</Link>
</div>
<div className='uk-navbar-right uk-flex'>
<ul className='uk-navbar-nav'>
<li className='uk-margin-right'>
{token
? <Link to='/login' onClick={() => { clearStorage('token'); clearStorage('books') }}>Logout</Link>
: <Link to='/login'>Login</Link>}
</li>
{/* this value is hard-coded, but eventually I will pass
username as a prop to this component from App.js */}
<li className='uk-margin-right'><Link to='/dashboard'>Hello, username!</Link></li>
</ul>
</div>
</nav>
)
}
<file_sep>/src/components/Booklist.js
import { useEffect, useState } from 'react'
import { Link } from 'react-router-dom'
import { BookForm } from './BookForm'
import { Book } from './Book'
import axios from 'axios'
import _ from 'lodash'
export const Booklist = ({ token, books, setBooks, featured, setFeatured }) => {
const [submitted, setSubmitted] = useState(false)
const [error, setError] = useState('')
const [search, setSearch] = useState('')
useEffect(() => {
axios.get('https://drf-library-api.herokuapp.com/api/books', {
headers: {
'Content-Type': 'application/json',
Authorization: `token ${token}`
}
}
).then((res) => setBooks(res.data))
}, [token, setBooks, submitted])
const handleSubmit = (e) => {
e.preventDefault()
if (search !== '') {
axios.get(`https://drf-library-api.herokuapp.com/api/books?search=${search}`, {
headers: {
'Content-type': 'application/json',
Authorization: `token ${token}`
}
}).then((res) => { setBooks(res.data); setSearch(''); setError('') })
}
return setError('Must add search term')
}
return (
<div className='
uk-cover-container
uk-flex-middle
uk-flex-column
uk-margin'
>
<input
type='text'
className='uk-input uk-margin-bottom'
placeholder='search by title'
value={search}
onChange={(e) => setSearch(e.target.value)}
/>
<button type='submit' className='uk-button' onClick={e => handleSubmit(e)}>Search</button>
{error !== '' ? <p style={{ color: 'red' }}>{error}</p> : null}
{books && !_.isEmpty(books)
? books.map((book) => {
return (
<div key={book.pk} className='uk-flex uk-flex-column uk-align-center uk-width-1-2@m uk-card uk-card-default'>
<div className='uk-card-body'>
{book.featured && <>Featured <i className='fas fa-trophy uk-margin-left' /></>}
<Book book={book} pk={book.pk} token={token} featured={featured} setFeatured={setFeatured} />
<Link to={`/books/${book.pk}`}>View Details</Link>
</div>
</div>
)
})
: <div>No results found</div>}
{token && <BookForm token={token} setBooks={setBooks} setSubmitted={setSubmitted} />}
</div>
)
}
<file_sep>/src/App.js
import { useState } from 'react'
import { Login } from './components/Login'
import { Booklist } from './components/Booklist'
import { Navbar } from './components/Navbar'
import { Register } from './components/Register'
import { Book } from './components/Book'
import { Dashboard } from './components/Dashboard'
import { BrowserRouter as Router, Route, Switch, Redirect } from 'react-router-dom'
import useLocalStorageState from 'use-local-storage-state'
function App () {
const [auth, setAuth, { removeItem }] = useLocalStorageState('token', '')
const [books, setBooks] = useLocalStorageState('books', [])
const [featured, setFeatured] = useState(false)
return (
<Router>
<Navbar token={auth} clearStorage={removeItem} />
<Switch>
<Route path='/login' component={() => <Login setAuth={setAuth} />} />
<Route path='/register' component={() => <Register setAuth={setAuth} />} />
<Route
exact path='/' render={() => auth
? <Booklist token={auth} books={books} setBooks={setBooks} featured={featured} setFeatured={setFeatured} />
: <Redirect to={{ pathname: '/login' }} />}
/>
<Route path='/books/:id' component={(id) => <Book token={auth} pk={id} featured={featured} setFeatured={setFeatured} />} />
<Route path='/dashboard' component={Dashboard} />
</Switch>
</Router>
)
}
export default App
<file_sep>/src/components/Login.js
import { useState } from 'react'
import { useHistory, Link } from 'react-router-dom'
import axios from 'axios'
export const Login = ({ setAuth }) => {
const [password, setPassword] = useState('')
const [username, setUsername] = useState('')
const history = useHistory()
const handleSubmit = (event) => {
event.preventDefault()
axios.post('https://drf-library-api.herokuapp.com/auth/token/login',
{
username: username,
password: <PASSWORD>
})
.then(res => {
if (res.data.auth_token) {
setAuth(res.data.auth_token)
history.push('/')
}
return null
})
}
const handleChange = (inputType, event) => {
if (inputType === 'username') {
setUsername(event.target.value)
}
if (inputType === 'password') {
setPassword(event.target.value)
}
}
return (
<div className='uk-container uk-flex uk-flex-center uk-flex-middle uk-height-large'>
<form className='uk-form-horizontal' onSubmit={handleSubmit}>
<label className='uk-form-label'>Username</label>
<input
className='uk-input'
type='text'
placeholder='<EMAIL> or janedoge123'
value={username}
onChange={(e) => handleChange('username', e)}
/>
<label className='uk-form-label'>Password</label>
<input
className='uk-input uk-margin-bottom'
placeholder='<PASSWORD>'
type='<PASSWORD>'
// using state to pass a value to this attribute
// makes this a controlled component
value={password}
onChange={(e) => handleChange('password', e)}
/>
<div id='form-buttons'>
<button
className='uk-button uk-margin-right'
type='submit'
>Login
</button>
<span>New to Bookify? <Link to='/register'> <a className='uk-link uk-primary' href='#'>Register Now</a></Link></span>
</div>
</form>
</div>
)
}
<file_sep>/src/components/Book.js
import axios from 'axios'
import { useLocation } from 'react-router-dom'
import { useEffect, useState } from 'react'
export const Book = ({ book, token, pk, featured, setFeatured }) => {
const [selectedBook, setSelectedBook] = useState(book)
const location = useLocation()
const bookDetails =
(selectedBook &&
<div className='uk-flex uk-cover-container uk-flex-column'>
<h3 className='uk-text-center'>{selectedBook.title}</h3>
</div>
)
const handleFeatured = (e) => {
e.preventDefault()
axios.patch(`http://drf-library-api.herokuapp.com/api/books/${selectedBook.pk}`, {
featured: !selectedBook.featured
},
{
headers: {
Authorization: `token ${token}`
}
}
).then(res => { setFeatured(!featured) })
}
useEffect(() => {
if (location.pathname.includes('/books/')) {
axios.get(`https://drf-library-api.herokuapp.com/api/books/${pk.match.params.id}`, {
headers: {
Authorization: `token ${token}`
}
})
.then((res) => {
setSelectedBook(res.data)
})
}
}, [location.pathname, pk, token])
return (
<div>
{location.pathname === '/'
? <h3>{selectedBook.title}</h3>
: <>
{bookDetails}
<button className='uk-button uk-align-center' onClick={handleFeatured}>
{selectedBook && selectedBook.featured ? 'Remove From Featured' : 'Add To Featured'}
</button>
</>}
</div>
)
}
| dbed881c508052370589df467e49684bb23457ae | [
"JavaScript"
] | 6 | JavaScript | Momentum-Team-9/react-router-example | cc00739034dde38da93883f95bafedebe07e10a6 | 4ec7519f0b2463c7f4736fd78bf7828d2d715572 |
refs/heads/main | <repo_name>egonw/bioversions<file_sep>/tests/test_bioversions.py
# -*- coding: utf-8 -*-
"""Tests for bioversions."""
import datetime
import unittest
import bioversions
from bioversions.sources import BioGRIDGetter, WikiPathwaysGetter
class TestGetter(unittest.TestCase):
"""Tests for the Getter class."""
def test_get(self):
"""Test getters."""
prefixes = [
"reactome",
"kegg",
]
for prefix in prefixes:
with self.subTest(prefix=prefix):
s = bioversions.get_version(prefix)
self.assertIsInstance(s, str)
def test_getter(self):
"""Test the BioGRID getter."""
s = BioGRIDGetter.version
self.assertIsInstance(s, str)
def test_date(self):
"""Test getters that have versions as dates."""
for getter in [WikiPathwaysGetter]:
with self.subTest(getter=getter.name):
s = getter.version
self.assertIsInstance(s, str)
d = getter.version_date_parsed
self.assertIsInstance(d, datetime.date)
| ebc258dd9fa0969772c8e367edd697b57d159d2e | [
"Python"
] | 1 | Python | egonw/bioversions | 879b40fe3998ece884d4ace1fc53eb524b74635b | af15cd2d29662248092476e6a9433f38afc1aace |
refs/heads/master | <file_sep><?php
$servername = "localhost";
$username = "root";
$password = "<PASSWORD>";
$dbname = "test_index";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
for ($i=1; $i <= 100000 ; $i++) {
$sql = "INSERT INTO product (name, cat_id, partner_id, region_id, description)
VALUES (CONCAT('Product-', ".$i."), ROUND((RAND() * (100-1))+1), ROUND((RAND() * (100-1))+1), ROUND((RAND() * (100-1))+1), MD5(ROUND((RAND() * (3000-1))+1)))";
if ($conn->query($sql) === TRUE) {
echo "New record created successfully";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
}
$conn->close();
?><file_sep><?php
$servername = "localhost";
$username = "root";
$password = "<PASSWORD>";
$dbname = "test_index";
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$data = '';
for($i = 1; $i < 10; $i++){
$data .= "('a', 1, '2', '3', 'ss'),";
}
$a = rtrim($data, ',');
$sql = "INSERT INTO product (name, cat_id, partner_id, region_id, description) VALUES {$a}";
$msc = microtime(true);
if ($conn->query($sql) === TRUE) {
$msc = microtime(true)-$msc;
echo "New record created successfully";
echo "Thoi gian thuc hien: ";
echo $msc . ' s'."<br/>"; // in seconds
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
| 3fb3925f5d4021518e04f7e780ea94527958a47f | [
"PHP"
] | 2 | PHP | quanghuy95github/01magento_requirejs | 79d96b1459b55a447fa5786d610f4c95ba3ba582 | abbc6d87777b2b21879b03546cea44ef8bb23993 |
refs/heads/master | <file_sep>
GradualIntSqrt
==============
Functions for efficiently producing the integer square root of numbers that change gradually.
Please read the `API documentation here`__
__ https://docs.rs/gradual_int_sqrt/
|build_status|_ |crates|_
.. |build_status| image:: https://travis-ci.org/rust-gradual_int_sqrt/gradual_int_sqrt.svg?branch=master
.. _build_status: https://travis-ci.org/rust-gradual_int_sqrt/gradual_int_sqrt
.. |crates| image:: http://meritbadge.herokuapp.com/gradual_int_sqrt
.. _crates: https://crates.io/crates/itertools
How to use with cargo:
.. code:: toml
[dependencies]
gradual_int_sqrt = "0.1"
How to use in your crate:
.. code:: rust
use gradual_int_sqrt;
License
-------
Dual-licensed to be compatible with the Rust project.
Licensed under the Apache License, Version 2.0
http://www.apache.org/licenses/LICENSE-2.0 or the MIT license
http://opensource.org/licenses/MIT, at your
option. This file may not be copied, modified, or distributed
except according to those terms.
<file_sep>//!
//! This crate contains functions that generate functions that calculate
//! the integer square root (hereafter "isqrt") of a number.
//! For example, the integer square root of 30 is 5, since 5^2 = 25 and
//! 6^2 = 36.
//! These generated functions are very efficient at processing gradually
//! changing sequences of numbers.
//! They achieve this efficiency by remembering the previous square root
//! calculated and making use of that in calculating the next square root.
//!
//! As a trivial example, if the last value processed was 133, then the
//! algorithm has figured out that the isqrt was 11, and that that value
//! is good up to 143. So if the next invocation asks for the isqrt of
//! 136, it simply returns the previous answer of 11 again. For a value
//! of 145 it would recognize that 11 is too low, add 2*12 + 1 to the
//! previous end value of 143 to get 168, and see that 12 is the isqrt.
//! Multiplying by 2 is just a trivial shift left, so as long as the
//! current value is not very far from the previous value, its isqrt
//! costs very little to produce. If, however, the next invocation
//! asks for the isqrt of 1,000,293, then it will take many iterations
//! to reach the correct isqrt value of 1000.
//!
//! Here is an example involving an ascending sequence:
//! ```
//! let to_isqrt = gradual_int_sqrt::int_sqrt_gradually_ascending_from::<u16, u8>(0);
//! let result: Vec<u8> = (0u16..17).map(to_isqrt).collect();
//! let expected: Vec<u8> = vec![
//! // 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 // n
//! 0, 1, 1, 1, 2, 2, 2, 2, 2, 3, 3, 3, 3, 3, 3, 3, 4 // isqrt(n)
//! ];
//! assert_eq!(result, expected);
//! ```
//!
//! Note that the input type and the isqrt type are separate. Care is taken
//! to ensure that the algorithm works without overflow for unsigned integers
//! as long as the type of the isqrt is at least half as wide as the type of
//! the inputs. For example, if the input type is u16, then the output type
//! can be u8 with no possibility of overflow.
//!
//! Generally speaking, this crate should only be used when the following
//! are all true:
//! 1. You have a lot of values, perhaps a continuous stream of them,
//! for which you need some measure of the square root.
//! 3. Either you really want the integer square root, or you want the
//! square root but don't need much precision.
//! 2. The input values do not jump around wildly.
//!
//! A good example of where this crate could help is in processing sensor
//! data in embedded systems that do not have floating-point units or even
//! fast integer division circuitry.
//! If you were to take, say, 32 or 64 samples
//! per second from an accelerometer or gyroscope in a hand-held device,
//! the values would rise and fall within some range as the sensors tracked
//! your movements, but would not jump all over the place.
//! You could use isqrt(x<sup>2</sup> + y<sup>2</sup>) as a rough measure
//! of the magnitude of the acceleration in the XY plane (for example).
//!
//! It is possible to increase precision by scaling the input values,
//! usually by a power of 2 to make it faster. For example, multiplying
//! incoming values by 64 improves the resolution of the function 8X.
//! This will have some performance cost, since the function will have
//! to search more to find the isqrt, especially near 0.
//!
//! ```
//! let mut to_isqrt = gradual_int_sqrt::int_sqrt_gradually_ascending_from::<u16, u8>(0);
//! let result: Vec<u8> = (0u16..17).map(|n| to_isqrt(64*n)).collect();
//! let expected: Vec<u8> = vec![
//! // 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 // n
//! // == // e.g. n = 15
//! // 0, 1, 1, 1, 2, 2, 2, 2, 2, 3, 3, 3, 3, 3, 3, 3, 4 // isqrt(15) = 3
//! 0, 8,11,13,16,17,19,21,22,24,25,26,27,28,29,30,32 // isqrt(15*64) = 30
//! // 30/8 = 3.75 is much closer to sqrt(15) than 3 is
//! ];
//! assert_eq!(result, expected);
//! ```
//!
//! There are several versions of the algorithm, allowing for extra
//! efficiency in situations where you know that the values are sorted
//! (or at least are not so unsorted that you would care).
//!
//! In cases where you know that a big jump has occurred (e.g. in
//! a reset function), you can simply regenerate the isqrt function
//! with an appropriate initial isqrt value.
//!
use std::ops::{Add, Sub, Mul, AddAssign, SubAssign};
use std::fmt::Debug;
/// Returns a function that calculates the integer square root of a number.
/// The returned function can very efficiently produce such a square root
/// if the input value is near the previous input value (or the init value,
/// if this is the first call).
/// ```
/// let to_isqrt = gradual_int_sqrt::int_sqrt_gradually_changing_from::<u16, u8>(0);
/// let result: Vec<u8> = (0u16..10).chain((0u16..10).rev())
/// .map(to_isqrt)
/// .collect();
/// let expected: Vec<u8> = vec![
/// //1 2 3 4 5 6 7 8 9 9 8 7 6 5 4 3 2 1 0 // n
/// 0,1,1,1,2,2,2,2,2,3,3,2,2,2,2,2,1,1,1,0 // isqrt(n)
/// ];
/// assert_eq!(result, expected);
/// ```
pub fn int_sqrt_gradually_changing_from<Num, Sqrt>(init: Sqrt) -> impl FnMut(Num) -> Sqrt where
Num: Debug + Add<Output = Num> + AddAssign + SubAssign + Copy + From<u8> + Sub<Output = Num> + Mul<Output = Num> + Ord,
Sqrt: Debug + Add<Output = Sqrt> + AddAssign + SubAssign + Copy + From<u8> + Into<Num>
{
let mut sqrt: Sqrt = init; // the current square root
let s: Num = init.into();
let mut lo: Num = s * s;
let mut hi: Num = lo + (s * 2.into()); // (s + 1)^2 - 1 without overflowing
move |n: Num| {
// If the current sqrt doesn't work for this n,
// increment it until it does.
if n > hi {
while n > hi {
sqrt += 1.into();
let s: Num = sqrt.into();
lo = hi + 1.into();
hi = lo + s + s;
}
} else {
while n < lo {
sqrt -= 1.into();
let s: Num = sqrt.into();
hi = lo - 1.into();
lo = hi - s - s;
}
}
sqrt
}
}
/// Returns a function that calculates the integer square root of a number.
/// The returned function can very efficiently produce such a square root
/// if the input value is near the previous input value (or the init value,
/// if this is the first call). This version assumes that it will be called
/// with increasing values; if you call it with a lower value, the previous
/// isqrt will be returned again.
/// ```
/// let to_isqrt = gradual_int_sqrt::int_sqrt_gradually_ascending_from::<u16, u8>(0);
/// let result: Vec<u8> = (0u16..10).map(to_isqrt).collect();
/// let expected: Vec<u8> = vec![
/// // 0 1 2 3 4 5 6 7 8 9 // n
/// 0, 1, 1, 1, 2, 2, 2, 2, 2, 3 // isqrt(n)
/// ];
/// assert_eq!(result, expected);
/// ```
pub fn int_sqrt_gradually_ascending_from<Num, Sqrt>(init: Sqrt) -> impl FnMut(Num) -> Sqrt where
Num: Debug + Add<Output = Num> + AddAssign + Copy + From<u8> + Mul<Output = Num> + Ord,
Sqrt: Debug + Add<Output = Sqrt> + AddAssign + Copy + From<u8> + Into<Num>
{
let mut sqrt: Sqrt = init; // the current square root
let s: Num = init.into();
let mut hi: Num = s * (s + 2.into()); // (s + 1)^2 - 1 without overflowing
move |n: Num| {
// If the current sqrt doesn't work for this n,
// increment it until it does.
while n > hi {
sqrt += 1.into();
let s: Num = sqrt.into();
hi += s + s + 1.into();
}
sqrt
}
}
/// Returns a function that calculates the integer square root of a number.
/// The returned function can very efficiently produce such a square root
/// if the input value is near the previous input value (or the init value,
/// if this is the first call). This version assumes that it will be called
/// with decreasing values; if you call it with a higher value, the previous
/// isqrt will be returned again.
/// ```
/// let to_isqrt = gradual_int_sqrt::int_sqrt_gradually_descending_from::<u16, u8>(5);
/// let result: Vec<u8> = (0u16..10).rev().map(to_isqrt).collect();
/// let expected: Vec<u8> = vec![
/// // 9 8 7 6 5 4 3 2 1 0 // n
/// 3, 2, 2, 2, 2, 2, 1, 1, 1, 0 // isqrt(n)
/// ];
/// assert_eq!(result, expected);
/// ```
pub fn int_sqrt_gradually_descending_from<Num, Sqrt>(init: Sqrt) -> impl FnMut(Num) -> Sqrt where
Num: Debug + Add<Output = Num> + SubAssign + Copy + From<u8> + Mul<Output = Num> + Ord,
Sqrt: Debug + Add<Output = Sqrt> + SubAssign + Copy + From<u8> + Into<Num>
{
let mut sqrt: Sqrt = init; // the current square root
let s: Num = init.into();
let mut lo: Num = s * s;
move |n: Num| {
// If the current sqrt doesn't work for this n,
// decrement it until it does.
while n < lo {
sqrt -= 1.into();
let s: Num = sqrt.into();
lo -= s + s + 1.into();
}
sqrt
}
}
///////////////////////////////////////////////////////////////////////////
#[cfg(test)]
extern crate more_asserts;
#[cfg(test)]
mod tests {
use super::*;
use more_asserts::*;
#[test]
fn test_asc_u16_u8() {
let to_isqrt = int_sqrt_gradually_ascending_from::<u16, u8>(0);
let result: Vec<u8> = (0u16..10)
.map(to_isqrt)
.collect();
let expected: Vec<u8> = vec![
// 1 2 3 4 5 6 7 8 9
0, 1, 1, 1, 2, 2, 2, 2, 2, 3
];
assert_eq!(result, expected);
}
#[test]
fn test_entire_asc_range_u16_u8() {
let mut to_isqrt = int_sqrt_gradually_ascending_from::<u16, u8>(0);
for n in 0u16..65535 { // For every possible u16 value ...
let s = to_isqrt(n);
let t = s as u32;
let n = n as u32;
assert_le!(t*t, n); // the isqrt is not too high: isqrt(n)^2 <= n
let t = t + 1;
assert_gt!(t*t, n); // the isqrt is high enough: (isqrt(n) + 1)^2 > n
}
}
#[test]
fn test_asc_u16_u16() {
let to_isqrt = int_sqrt_gradually_ascending_from::<u16, u16>(0);
let result: Vec<_> = (0u16..10)
.map(to_isqrt)
.collect();
let expected: Vec<u16> = vec![
0, 1, 1, 1, 2, 2, 2, 2, 2, 3
];
assert_eq!(result, expected);
}
#[test]
fn test_asc_u32_u16() {
let to_isqrt = int_sqrt_gradually_ascending_from::<u32, u16>(0);
let result: Vec<_> = (0u32..10)
.map(to_isqrt)
.collect();
let expected: Vec<u16> = vec![
0, 1, 1, 1, 2, 2, 2, 2, 2, 3
];
assert_eq!(result, expected);
}
#[test]
fn test_asc_i32_i16() {
// Overflow is possible with i32_i16, but not with these numbers.
let to_isqrt = int_sqrt_gradually_ascending_from::<i32, i16>(0);
let result: Vec<_> = (0i32..10)
.map(to_isqrt)
.collect();
let expected: Vec<i16> = vec![
0, 1, 1, 1, 2, 2, 2, 2, 2, 3
];
assert_eq!(result, expected);
}
#[test]
fn test_scaled_asc_u32_u16() {
let mut to_isqrt = int_sqrt_gradually_ascending_from::<u32, u16>(0);
let result: Vec<_> = (0u32..10)
.map(|n| to_isqrt(1024*n))
.collect();
let expected: Vec<u16> = vec![
0, 32, 45, 55, 64, 71, 78, 84, 90, 96
];
assert_eq!(result, expected);
}
#[test]
fn test_desc_u16_u8() {
let to_isqrt = int_sqrt_gradually_descending_from::<u16, u8>(5);
let result: Vec<u8> = (0u16..10)
.rev()
.map(to_isqrt)
.collect();
let expected: Vec<u8> = vec![
3, 2, 2, 2, 2, 2, 1, 1, 1, 0,
];
assert_eq!(result, expected);
}
#[test]
fn test_u16_u8() {
let to_isqrt = int_sqrt_gradually_changing_from::<u16, u8>(0);
let result: Vec<u8> = (0u16..10)
.chain((0u16..10).rev())
.map(to_isqrt)
.collect();
let expected: Vec<u8> = vec![
//1 2 3 4 5 6 7 8 9 9 8 7 6 5 4 3 2 1 0
0,1,1,1,2,2,2,2,2,3,3,2,2,2,2,2,1,1,1,0
];
assert_eq!(result, expected);
}
/*
// Float types don't implement Ord. Could make a PartialOrd version.
#[test]
fn test_f32_u16() {
let to_isqrt = int_sqrt_gradually_ascending_from::<f32, u16>(0);
let result: Vec<u16> = (0f32..10f32).map(to_isqrt).collect();
let expected: Vec<u16> = vec![
//1 2 3 4 5 6 7 8 9 9 8 7 6 5 4 3 2 1 0
0,1,1,1,2,2,2,2,2,3,3,2,2,2,2,2,1,1,1,0
];
assert_eq!(result, expected);
}
*/
}
| 9b8d390189b55819f9a07b3493315fc0f22fc3e1 | [
"Rust",
"reStructuredText"
] | 2 | reStructuredText | NicholasSterling/gradual_int_sqrt | 7ba0e84846fd79ad0eb8330c6b9e538e9d25429b | c3164c9108642f8a7bba173d4ca281e825d66a2a |
refs/heads/master | <repo_name>PabloK/Sinatra-springboard<file_sep>/config/helpers.rb
class Sinatra::Base
# Configure sinatra helpers here
def helper(user)
return nil
end
end
<file_sep>/config/globals.rb
$CONFIG = {}
$CONFIG[:test_key] = "test"
<file_sep>/spec/test/first_spec.rb
require './spec/spec_helper'
describe MainController, :type => :request do
it 'Renders Hello world' do
visit '/'
page.should have_content 'Hello world'
end
end
<file_sep>/config/environment.rb
require 'active_support/core_ext/numeric/time'
require 'active_support/core_ext/date_time/calculations'
require 'rubygems'
require 'sinatra/base'
require 'sinatra/flash'
require 'data_mapper'
require 'pony'
require 'uglifier'
class Sinatra::Base
# Production settings for haml
if ENV['RACK_ENV'] == 'production'
set :haml, { :ugly => true }
set :clean_trace, true
end
# Global-variable configuration
require './config/globals'
# View dir configuration
set :views, File.dirname(__FILE__) + "/../views"
# Register session based flash
register Sinatra::Flash
# Mailer configuration
options = {
:from => "<EMAIL>",
:via => :smtp,
:via_options => {
:user_name => ENV['SENDGRID_USERNAME'],
:password => ENV['<PASSWORD>'],
:domain => "localhost",
:address => "smtp.sendgrid.net",
:port => 587,
:authentication => :plain,
:enable_starttls_auto => true
}
}
Pony.options = options
end
# SQL logging settings
if ENV['RACK_ENV'] == 'development'
DataMapper::Logger.new($stdout, :debug)
end
DataMapper::setup(:default, ENV['DATABASE_URL'])
<file_sep>/README.md
Sinatra-springboard
===================
This application is an attempt at creating a basic starting point for app development of full-stack applications. It will try to generalize as much as possible but in specifics will cater to my personal development needs. I will try to keep the springboard as easy as possible to install to begin with ruby 1.9+, rubygems and bundler are required. A few gems will be installed during by bundler aswell these gems have some external dependancies that are not hard to come over trough apt-get or similar.
##Installation
```bash
git clone https://github.com/PabloK/Sinatra-springboard.git
cd Sinatra-springboard
bundle install
```
##Running the server
```bash
rackup
```
##Usage
Place your controlers in lib/controlers and map them to a url in the config.ru file. Data models are placed in the lib models directory and can be used to create presistant storage using the datamapper API. To use this you need to configure a database and modify database configuration in config/environment.rb. Email is also configurable in environment.rb. Global variables can be configured in config/globals.conf. Sinatra helpers can be configured in conf/helpers.rb. Tests are executed trough the use of the following command
```bash
rake spec
```
Tests are located in separate folders inside the specfolder. Tests are configured in the spec/spec_helper.rb file. Html views are placed in the views directory where the main layout file layout.haml also exists.
Coffeescript & Sass files are converted by their respective controlers found in the lib/controler folders. .coffee and .sass files belong in the assets/js & assets/css folders respectivley.
Configuration of publicfolders is done in the config.ru file and starts with configuring audio and images to be located in the public folder in respective subfolder. robots.txt is also configured here.
For full feature the following environment variables needs to be configured. Preferably in your .bashrc file.
```bash
# Postgres is just an example and comes preconfigured some changes are needed in conf/environment.rb to use MYSQL or Sqlite
export DATABASE_URL="postgres://user:password@host/datbase"
export SESSION_SECRETE=""
#The following is just for sendgrid users
export SENDGRID_PASSWORD=""
export SENDGRID_USERNAME=""
```
<file_sep>/config.ru
require './config/environment'
require './config/helpers'
require './lib/controllers'
map '/' do
# Rack configuration for audio and images
use Rack::Static, {
:root => "public",
:urls => ["/audio"],
:content_type => "audio/mpeg",
:cache_control => "public,max-age=#{365 * 24 * 3600}"
}
use Rack::Static, {
:root => "public",
:urls => ["/img"],
:cache_control => "public,max-age=#{365 * 24 * 3600}"
}
use Rack::Static, {
:root => "public",
:urls => ["/robots.txt"],
:cache_control => "public,max-age=#{365 * 24 * 3600}"
}
use Rack::Session::Cookie, :secret => ENV['SESSION_SECRETE'], :expire_after => 30 * 3600
# Configuration of css and javascript compilation
map '/css' do
run SassCssConverter
end
map '/js' do
run CoffeeJsConverter
end
map '/' do
run MainController
end
end
| b3e5f391fe90a07a45749dd54c90b4b6fc824a2d | [
"Markdown",
"Ruby"
] | 6 | Ruby | PabloK/Sinatra-springboard | fa80bf186dd40a33daa26bc841bf04d4ffa0f3a9 | e6ecf766f2d4085826f282fc34e78693cf6e60cb |
refs/heads/master | <repo_name>ah-kevin/Foot_App<file_sep>/Font_App/Restaurant.swift
//
// File.swift
// Font_App
//
// Created by 柯秉钧 on 16/6/28.
// Copyright © 2016年 柯秉钧. All rights reserved.
//
import Foundation
struct Restaurant {
var name: String
var type: String
var location: String
var image: String
var isVisited: Bool
var rating: String = ""
init(name: String, type: String, location: String, image: String, isVisited: Bool) {
self.name = name
self.type = type
self.location = location
self.image = image
self.isVisited = isVisited
}
}
| c8d20fd49b5d1a1660f648536595cf4e4312b06f | [
"Swift"
] | 1 | Swift | ah-kevin/Foot_App | 482dc6cf7199c00a9cc05ef90e8a9960f0d994ef | c1d902b9ab0cae736fbeb934d8fabfadc5954a77 |
refs/heads/master | <repo_name>morganfox612/Character_Counter<file_sep>/main.rb
$has = {}
puts "Please enter a string"
$count = 0
$char
$string = gets.chomp.upcase.gsub(/\s+/,"")
$x = $string.split('')
def most_often
$x.each do |y|
arr = $string.scan(y)
$count = arr.length
hash = {"#{y}": $count}
$has.merge!(hash)
end
puts $string
puts $has
end
most_often
| e369ce3be1d60f2a07152902a7371b5566f534e8 | [
"Ruby"
] | 1 | Ruby | morganfox612/Character_Counter | 5a0bad6764d70a52fb46e7054095e4fc70502c5a | 656e7d0822e7517b711281f459bf3f59ef33303b |
refs/heads/master | <repo_name>thelumiereguy/Threejs_Demo<file_sep>/src/script.js
import './style.css'
import * as THREE from 'three'
import * as dat from 'dat.gui'
const loader = new THREE.TextureLoader()
const texture = loader.load('/texture.png')
const displacement = loader.load('/DisplacementMap.png')
// Debug
const gui = new dat.GUI()
// Canvas
const canvas = document.querySelector('canvas.webgl')
// Scene
const scene = new THREE.Scene()
// Objects
const geometry = new THREE.PlaneBufferGeometry(5, 5, 256, 256)
// Materials
const materials = new THREE.MeshStandardMaterial({
color: 'gray',
map: texture,
displacementMap: displacement,
displacementScale: 2
})
// Mesh
const plane = new THREE.Mesh(geometry, materials)
scene.add(plane)
plane.rotation.x = 6
// plane.rotation.z = 43
gui.add(plane.rotation, 'x')
// Lights
const pointLight = new THREE.PointLight('#5244ea', 7)
pointLight.position.x = 4.4
pointLight.position.y = 30
pointLight.position.z = 1
scene.add(pointLight)
gui.add(pointLight.position, 'x')
gui.add(pointLight.position, 'y')
gui.add(pointLight.position, 'z')
const baseColor = {color: '#5244ea'}
gui.addColor(baseColor, 'color').onChange(() => {
pointLight.color.set(baseColor.color)
})
/**
* Sizes
*/
const sizes = {
width: window.innerWidth,
height: window.innerHeight
}
window.addEventListener('resize', () => {
// Update sizes
sizes.width = window.innerWidth
sizes.height = window.innerHeight
// Update camera
camera.aspect = sizes.width / sizes.height
camera.updateProjectionMatrix()
// Update renderer
renderer.setSize(sizes.width, sizes.height)
renderer.setPixelRatio(Math.min(window.devicePixelRatio, 2))
})
/**
* Camera
*/
// Base camera
const camera = new THREE.OrthographicCamera(-2, 2, 3, 0, 1, 50)
camera.position.x = 0
camera.position.y = 0
camera.position.z = 10
scene.add(camera)
gui.add(camera.rotation, 'x')
gui.add(camera.rotation, 'y')
gui.add(camera.rotation, 'z')
// Controls
// const controls = new OrbitControls(camera, canvas)
// controls.enableDamping = true
/**
* Renderer
*/
const renderer = new THREE.WebGLRenderer({
canvas: canvas,
alpha: true
})
renderer.setSize(sizes.width, sizes.height)
renderer.setPixelRatio(Math.min(window.devicePixelRatio, 2))
/**
* Animate
*/
const clock = new THREE.Clock()
const PERIOD = 8; // loop every 8 calls to updateNumber
const SCALE = 255; // go between 0 and this
const tick = () => {
const elapsedTime = clock.getElapsedTime()
// Update objects
pointLight.position.y = Math.sin(elapsedTime) + 24
let value = (Math.sin(elapsedTime * 2 * Math.PI / PERIOD) * (SCALE / 2) + (SCALE / 2));
pointLight.color.setRGB(value / 255, value / 255, 234 / 255)
// Render
renderer.render(scene, camera)
// Call tick again on the next frame
window.requestAnimationFrame(tick)
}
tick() | 05326ce6dd23bba4fcb8d5951a0ee162a2231f8f | [
"JavaScript"
] | 1 | JavaScript | thelumiereguy/Threejs_Demo | b9a458822e61fc9a09908d3f5d95f80ffa245985 | 5618695e9958f7f71b405e91571987de9950c751 |
refs/heads/master | <file_sep>var mongoose = require("mongoose");
var Campground = require("./models/campground");
var Comment = require("./models/comment");
var data =[
{
name:"Imoto",
image: "http://www.otakuusamagazine.com/wp-content/uploads/2017/12/eromanga.jpg",
description: "Kawai Kawai Kawai"
},
{
name:"Boruto",
image: "https://miscmedia-9gag-fun.9cache.com/images/thumbnail-facebook/1557216671.5403_tunyra_n.jpg",
description: "dsadsadjaksldjklsad"
},
{
name:"Imoto2",
image: "https://animeforever.org/wp-content/uploads/2018/06/Latomorihburlada.jpg",
description: "fsfsfdsfdvcxvcxvxcvx"
}
]
function seedDB(){
Campground.remove({},function(err){
if(err){
console.log(err);
}
console.log("Removed");
//add a few campgrounds
data.forEach(function(seed){
Campground.create(seed,function(err,campground){
if(err){
console.log(err)
} else {
console.log ("added a campground");
//add a few comments
Comment.create(
{
text:"This is so cute",
author: "Me"
},function(err,comment){
if(err){
console.log(err);
} else{
campground.comments.push(comment);
campground.save();
console.log("created new comment")
}
});
}
});
});
});
}
module.exports = seedDB;
<file_sep># Try Here!!
https://secure-bayou-49094.herokuapp.com/
# AnimeCamp - Web App
It is just a personal project which is a website that you can signup and login to share anime pictures.
Also, you can comment and edit it.
| 1f09e568cc08123b13aa5f172eb65741c7b180c8 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | Critsu/AnimeCamp | 2227f9b2da5d0e72fe8c5bc62ab3b5389d6cdba8 | 3439878b51495bace647938a5bd3b8159fbb427f |
refs/heads/main | <file_sep>from django.urls import path
from chat_app.views import ChatRequestView, SetWorkStatusView, SetCloseStatusView
urlpatterns = [
path('<int:pk>/', ChatRequestView.as_view(), name='chat_request'),
path('<int:pk>/set_work', SetWorkStatusView.as_view(), name='set_status_work'),
path('<int:pk>/set_close', SetCloseStatusView.as_view(), name='set_status_close'),
]
<file_sep>from django.db import models
from requests_app.models import Request
class RequestMessage(models.Model):
STATUS_CHOICES = [
('отправлено', 'отправлено'),
('доставлено', 'доставлено'),
]
request = models.ForeignKey(Request, on_delete=models.CASCADE, verbose_name='Заявка')
is_system = models.BooleanField(default=False)
status = models.CharField(max_length=20, choices=STATUS_CHOICES, verbose_name='Статус сообщения')
content = models.CharField(max_length=500, verbose_name='Контент')
date = models.DateTimeField(auto_now_add=True, verbose_name='Дата, время отправки сообщения')
def __str__(self):
return self.content
class Attachment(models.Model):
message = models.ForeignKey(RequestMessage, on_delete=models.CASCADE)
file = models.FileField(upload_to="images/requests/attachment_message/", blank=True, null=True)
<file_sep>import re
from django import forms
from django.contrib.auth.forms import UserCreationForm
from django.contrib.auth.models import User
from django.forms import ModelForm
from django.utils.translation import ugettext_lazy as _
from .constants import HELP_TEXT_NUMBER_PHONE, PATTERN_NUMBER_PHONE
from .models import OrganisationProfile
class ExtendedAccountCreationForm(UserCreationForm):
class Meta:
model = User
fields = ['username', 'email', 'first_name', 'last_name', '<PASSWORD>', '<PASSWORD>']
labels = {
'username': _('Придумайте логин'),
'email': _('Электронная почта'),
'first_name': _('Имя'),
'last_name': _('Фамилия'),
}
def save(self, commit=True):
user = super().save(commit=False)
user.email = self.cleaned_data['email']
user.first_name = self.cleaned_data['first_name']
user.last_name = self.cleaned_data['last_name']
if commit:
user.save()
return user
class OrganisationProfileForm(ModelForm):
class Meta:
model = OrganisationProfile
fields = ('name', 'address', 'phone_number')
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.fields['phone_number'].help_text = HELP_TEXT_NUMBER_PHONE
def clean(self):
cleaned_data = super(OrganisationProfileForm, self).clean()
phone_number = cleaned_data.get('phone_number')
if re.match(PATTERN_NUMBER_PHONE, phone_number) is None:
raise forms.ValidationError(f'{phone_number} не соответствует примеру телефона')
return self.cleaned_data
<file_sep>{% extends 'base.html' %}
{% load crispy_forms_tags %}
{% block title %}
Регистрация
{% endblock title %}
{% block content %}
<h1 class="text-center">Регистрация нового аккаунта</h1>
<form method="POST" action="{% url 'register' %}">
{% csrf_token %}
<div class="jumbotron">
<h1>Информация об аккаунте</h1>
{{ form|crispy }}
</div>
<div class="jumbotron">
<h1>Информация об организации</h1>
{{ profile_form|crispy }}
</div>
<button type="submit" class="btn btn-danger btn-block">Зарегистрироваться</button>
</form>
{% endblock content %}<file_sep>FROM python:3.8
ENV PYTHONUNBUFFERED 1
RUN mkdir -p /usr/src/app
WORKDIR /usr/src/app
COPY . /usr/src/app
RUN pip install --no-cache-dir -r requirements.txt
RUN python manage.py makemigrations
RUN python manage.py migrate --noinput
RUN python manage.py initadmin
EXPOSE 8000
CMD ["python", "manage.py", "runserver", "0.0.0.0:8000"]<file_sep>from django.conf import settings
from django.contrib.auth.models import User
from django.core.management.base import BaseCommand
class Command(BaseCommand):
def handle(self, *args, **options):
invalidInputs = ["", None]
if User.objects.count() == 0:
for user in settings.ADMINS:
username = user[0].replace(' ', '')
email = user[1]
password = <PASSWORD>[2]
if username.strip() in invalidInputs or password.strip() in invalidInputs:
return None
user = User(
username=username,
email=email,
)
user.set_password(password)
user.is_superuser = True
user.is_staff = True
user.save()
else:
print('Admin accounts can only be initialized if no Accounts exist')
<file_sep>HELP_TEXT_NUMBER_PHONE = 'Примеры ввода телефонов: 89090951670, +79090951670, +7-910-221-22-22, +7(910)-221-22-22'
PATTERN_NUMBER_PHONE = '^\+?[78][-\(]?\d{3}\)?-?\d{3}-?\d{2}-?\d{2}$'<file_sep>from django.core import validators
from django.db import models
from accounts_app.models import OrganisationProfile
class Request(models.Model):
TYPE_REQUESTS_CHOICES = [
('Электрика', 'Электрика'),
('Сантехника', 'Сантехника'),
('Вентиляция', 'Вентиляция'),
('Связь и наблюдение', 'Связь и наблюдение'),
('Плотнические работы', 'Плотнические работы'),
]
STATUS_CHOICES = [
('На рассмотрении', 'На рассмотрении'),
('В работе', 'В работе'),
('Закрыта', 'Закрыта'),
]
type = models.CharField(max_length=30, verbose_name='Тип заявки', choices=TYPE_REQUESTS_CHOICES)
title = models.CharField(max_length=100, verbose_name='Краткое описание проблемы')
description = models.TextField(verbose_name='Подробности проблемы')
number_office = models.CharField(verbose_name='Номер офиса', max_length=5)
image = models.ImageField(verbose_name='Фото проблемы', upload_to="images/requests/main_pictures/",
validators=[validators.validate_image_file_extension])
date_creation = models.DateTimeField(auto_now_add=True, verbose_name='Дата и время заявки')
account = models.ForeignKey(OrganisationProfile, on_delete=models.CASCADE)
status = models.CharField(choices=STATUS_CHOICES, default='На рассмотрении', verbose_name='Статус заявки',
max_length=25)
def __str__(self):
return f'{self.id}: {self.title}'
<file_sep>from django.urls import path
from accounts_app.views import RegisterView, LoginAccountView
urlpatterns = [
path('register/', RegisterView.as_view(), name='register'),
path('login/', LoginAccountView.as_view(), name='login'),
]
<file_sep>asgiref==3.3.4
Django==3.2.4
pytz==2021.1
sqlparse==0.4.1
django-crispy-forms
pillow
django-axes
django-role-permissions
python-dotenv<file_sep>from django.contrib.auth.forms import AuthenticationForm
from django.contrib.auth.views import LoginView
from django.shortcuts import render, redirect
from django.views import View
from .forms import ExtendedAccountCreationForm, OrganisationProfileForm
class RegisterView(View):
def get(self, request):
form = ExtendedAccountCreationForm()
profile_form = OrganisationProfileForm()
return render(request, 'registration.html', {'form': form, 'profile_form': profile_form})
def post(self, request):
form = ExtendedAccountCreationForm(request.POST)
profile_form = OrganisationProfileForm(request.POST)
if form.is_valid() and profile_form.is_valid():
account = form.save()
profile = profile_form.save(commit=False)
profile.representative = account
profile.save()
return redirect('login')
else:
return render(request, 'registration.html', {'form': form, 'profile_form': profile_form})
class LoginAccountView(LoginView):
form_class = AuthenticationForm
template_name = 'login.html'
class HomepageView(View):
def get(self, request):
return render(request, 'home.html')
<file_sep>IMAGE_HELP_TEXT = 'Загружайте изображения с минимальны разрешенем 400x400.<br>' \
'Загружайте изображения с минимальны разрешенем 2000x2000.<br>' \
'Размер максимально загружаемого изображения не должен превышать 5 Мб.'
MIN_RESOLUTION_IMAGE = (400, 400)
MAX_RESOLUTION_IMAGE = (2000, 2000)
MAX_SIZE_BYTES_IMAGE = 5242880
<file_sep>from rolepermissions.roles import AbstractUserRole
class Manager(AbstractUserRole):
available_permissions = {
'manage_requests': True,
}
<file_sep>import os
from pathlib import Path
from datetime import timedelta
from dotenv import load_dotenv
# загрузка файла c переменными среды .env
env_path = Path('.')/'.env'
load_dotenv(dotenv_path=env_path)
BASE_DIR = Path(__file__).resolve().parent.parent
SECRET_KEY = os.getenv("SECRET_KEY")
DEBUG = bool(os.getenv("DEBUG"))
ALLOWED_HOSTS = [os.getenv("HOST")]
THIRD_PARTY_APPS = ['crispy_forms', 'axes', 'rolepermissions', ]
MY_APPS = ['accounts_app', 'requests_app', 'chat_app']
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
] + THIRD_PARTY_APPS + MY_APPS
MIDDLEWARE = [
'django.middleware.security.SecurityMiddleware',
'django.contrib.sessions.middleware.SessionMiddleware',
'django.middleware.common.CommonMiddleware',
'django.middleware.csrf.CsrfViewMiddleware',
'django.contrib.auth.middleware.AuthenticationMiddleware',
'django.contrib.messages.middleware.MessageMiddleware',
'django.middleware.clickjacking.XFrameOptionsMiddleware',
'axes.middleware.AxesMiddleware',
]
ROOT_URLCONF = 'management_company_interaction_project.urls'
AUTHENTICATION_BACKENDS = [
'axes.backends.AxesBackend',
'django.contrib.auth.backends.ModelBackend',
]
CRISPY_TEMPLATE_PACK = 'bootstrap4'
TEMPLATE_DIR = os.path.join(BASE_DIR, 'templates')
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [TEMPLATE_DIR],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
WSGI_APPLICATION = 'management_company_interaction_project.wsgi.application'
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': BASE_DIR / 'db.sqlite3',
}
}
AUTH_PASSWORD_VALIDATORS = [
{
'NAME': 'django.contrib.auth.password_validation.UserAttributeSimilarityValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.MinimumLengthValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.CommonPasswordValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.NumericPasswordValidator',
},
]
LANGUAGE_CODE = 'ru-RU'
TIME_ZONE = 'UTC'
USE_I18N = True
USE_L10N = True
USE_TZ = True
# Static files (CSS, JavaScript, Images)
STATIC_URL = '/static/'
STATICFILES_DIRS = [
BASE_DIR / "static",
'/var/www/static/',
]
DEFAULT_AUTO_FIELD = 'django.db.models.BigAutoField'
MEDIA_URL = '/media/'
MEDIA_ROOT = BASE_DIR / 'media'
LOGIN_REDIRECT_URL = 'create_request'
LOGOUT_REDIRECT_URL = 'login'
# axes app's settings
AXES_FAILURE_LIMIT = int(os.getenv("AXES_FAILURE_LIMIT"))
AXES_LOCK_OUT_AT_FAILURE = True
AXES_COOLOFF_TIME = timedelta(seconds=int(os.getenv("AXES_COOLOFF_TIME")))
# role permissions app's settings
ROLEPERMISSIONS_MODULE = 'management_company_interaction_project.roles'
ROLEPERMISSIONS_REGISTER_ADMIN = True
# these settings are needed when running in docker (creating a superuser)
ADMINS = (
(os.getenv("ADMIN_LOGIN"), os.getenv("ADMIN_EMAIL"), os.getenv("ADMIN_PASS"))
)
<file_sep>from django.contrib import admin
from chat_app.models import RequestMessage
admin.site.register(RequestMessage)
<file_sep>from django.conf import settings
from django.conf.urls.static import static
from django.contrib import admin
from django.urls import path, include
from accounts_app.views import HomepageView
urlpatterns = [
path('admin/', admin.site.urls),
path('accounts/', include('accounts_app.urls')),
path('accounts/', include('django.contrib.auth.urls')),
path('request/', include('requests_app.urls')),
path('request/', include('chat_app.urls')),
path('', HomepageView.as_view(), name='home'),
]
if settings.DEBUG:
urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
<file_sep>from django.contrib import admin
from requests_app.models import Request
admin.site.register(Request)
<file_sep><!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"
integrity="<KEY>"
crossorigin="anonymous">
<script src="https://code.jquery.com/jquery-3.1.1.min.js" integrity="<KEY>
crossorigin="anonymous"></script>
{% block head_script %}
{% endblock head_script %}
<title>
{% block title %}
{% endblock title %}
</title>
</head>
<body>
<div class="d-flex flex-column flex-md-row align-items-center p-3 px-md-4 mb-3 bg-white border-bottom box-shadow">
<h3 class="my-0 mr-md-auto font-weight-normal"><a href="{% url 'home' %}">Управляющая компания</a></h3>
{% if user.is_authenticated %}
<nav class="my-2 my-md-0 mr-md-3">
<p class="p-2 text-dark">Hi, {{ user.username }}!</p>
</nav>
<a class="btn btn-outline-primary" href="{% url 'create_request' %}">Создать заявку</a>
<a class="btn btn-outline-danger" href="{% url 'logout' %}">Выйти из аккаунта</a>
{% endif %}
</div>
<div class="container">
<div class="row justify-content-center">
<div class="col-12">
{% block content %}
{% endblock content %}
</div>
</div>
</div>
{% block body_script %}
{% endblock body_script %}
</body>
</html><file_sep>from django.contrib.auth.mixins import LoginRequiredMixin
from django.db.models import Q
from django.shortcuts import render, redirect
from django.views import View
from accounts_app.models import OrganisationProfile
from requests_app.forms import NewRequestForm
from requests_app.models import Request
class CreateRequestView(LoginRequiredMixin, View):
def get(self, request):
form = NewRequestForm()
return render(request, 'create_request.html', {'form': form})
def post(self, request):
form = NewRequestForm(request.POST, request.FILES)
if form.is_valid():
new_request = form.save(commit=False)
new_request.account = OrganisationProfile.objects.get(representative=request.user)
new_request.save()
return redirect('chat_request', pk=new_request.pk)
else:
return render(request, 'create_request.html', {'form': form})
class ListRequestsView(LoginRequiredMixin, View):
def get(self, request):
account = OrganisationProfile.objects.get(representative=request.user)
requests = Request.objects.filter(account=account).order_by('date_creation').filter(
Q(status__icontains='На рассмотрении') | Q(status__icontains='В работе')
)
return render(request, 'list_requests.html', {'requests': requests})
<file_sep>from django.urls import path
from requests_app.views import CreateRequestView, ListRequestsView
urlpatterns = [
path('new/', CreateRequestView.as_view(), name='create_request'),
path('list/', ListRequestsView.as_view(), name='list_requests'),
]
<file_sep>from django.contrib import admin
# Register your models here.
from accounts_app.models import OrganisationProfile
admin.site.register(OrganisationProfile)<file_sep>$(document).on('submit','#set_work',function(e){
e.preventDefault();
$.ajax({
type:'POST',
url:$("#set_work").prop('action'),
data:{
csrfmiddlewaretoken:$('input[name=csrfmiddlewaretoken]').val(),
},
success: function(json){
if (json.status === 'OK'){
alert('on work');
$("#messages_area").append('<div class="alert alert-success">'+
'<p class="lead">'+ json.user +' <b>(менеджер)</b></p>' +
'<code>' + json.content + '</code><br>' +
'<kbd>' + json.date + '</kbd></div>');
$("#set_work").hide();
}
else{
alert('сообщение не отправлено');
}
},
error : function(xhr,errmsg,err) {
console.log(xhr.status + ": " + xhr.responseText);
}
});
});<file_sep>from PIL import Image
from django import forms
from django.forms import ModelForm
from requests_app.constants import IMAGE_HELP_TEXT, MIN_RESOLUTION_IMAGE, MAX_RESOLUTION_IMAGE, MAX_SIZE_BYTES_IMAGE
from requests_app.models import Request
class NewRequestForm(ModelForm):
class Meta:
model = Request
fields = ('type', 'title', 'description', 'number_office', 'image')
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.fields['image'].help_text = IMAGE_HELP_TEXT
def clean(self):
cleaned_data = super(NewRequestForm, self).clean()
image = cleaned_data.get('image')
if image is None:
raise forms.ValidationError('Вы загрузили не изображение')
img = Image.open(image)
min_height, min_width = MIN_RESOLUTION_IMAGE
if img.height < min_height or img.width < min_width:
raise forms.ValidationError('Разрешение изображения меньше минимального')
max_height, max_width = MAX_RESOLUTION_IMAGE
if img.height > max_height or img.width > max_width:
raise forms.ValidationError('Разрешение изображения больше максимального')
if image.size > MAX_SIZE_BYTES_IMAGE:
raise forms.ValidationError('Размер загруженного изображения больше 5 Мб')
return cleaned_data
<file_sep>from django.contrib.auth.mixins import LoginRequiredMixin
from django.http import JsonResponse
from django.shortcuts import render, redirect
from django.views import View
from chat_app.constants import CLOSE_STATUS_MESSAGE, WORK_STATUS_MESSAGE
from chat_app.models import RequestMessage
from requests_app.models import Request
from rolepermissions.checkers import has_permission
class ChatRequestView(LoginRequiredMixin, View):
def get(self, request, pk):
selected_request = Request.objects.get(pk=pk)
is_manager = has_permission(request.user, 'create_medical_record')
if selected_request.account.representative != request.user and not is_manager:
return redirect('list_requests')
messages = RequestMessage.objects.filter(request=selected_request)
return render(request, 'chat_request.html', {'selected': selected_request,
'messages': messages,
'is_manager': is_manager})
def post(self, request, pk):
response_data = {}
try:
selected_request = Request.objects.get(pk=pk)
content = request.POST.get('content')
new_message = RequestMessage.objects.create(
request=selected_request,
status='отправлено',
content=content
)
response_data['status'] = 'OK'
response_data['user'] = request.user.username
response_data['content'] = content
response_data['date'] = new_message.date.strftime("%d %B %Y г. %H:%M")
return JsonResponse(response_data)
except Exception as e:
print(e)
response_data['status'] = 'BAD'
return JsonResponse(response_data)
class SetWorkStatusView(View):
def post(self, request, pk):
response_data = {}
try:
response_data = get_response_data(pk, 'В работе', request, WORK_STATUS_MESSAGE)
return JsonResponse(response_data)
except Exception as e:
print(e)
response_data['status'] = 'BAD'
return JsonResponse(response_data)
class SetCloseStatusView(View):
def post(self, request, pk):
response_data = {}
try:
response_data = get_response_data(pk, 'Закрыта', request, CLOSE_STATUS_MESSAGE)
return JsonResponse(response_data)
except Exception as e:
print(e)
response_data['status'] = 'BAD'
return JsonResponse(response_data)
def get_response_data(id, status, request, content_msg):
data = {}
selected_request = Request.objects.get(pk=id)
selected_request.status = status
selected_request.save()
new_message = RequestMessage.objects.create(
request=selected_request,
status='отправлено',
content=content_msg,
is_system=True
)
data['status'] = 'OK'
data['user'] = request.user.username
data['content'] = content_msg
data['date'] = new_message.date.strftime("%d %B %Y г. %H:%M")
return data
<file_sep><h1 align="center">
ManagementCompanyWebServiceDjango
</h1>
# What is it?
The application is designed to facilitate the interaction of the management company with consumers via chat.
# How to start ?
First, we need to upload the project to our local machine. To do this, in the command console, enter:
```sh
https://github.com/AndrewErmakov/ManagementCompanyWebServiceDjango.git
```
Also you will have a `.env` file. This file must be in the same directory together `Dockerfile` and `manage.py`. You need to specify a nickname, mail and password for the administrator (ADMIN_LOGIN, ADMIN_EMAIL and ADMIN_PASS in `.env` file respectively).
**Alas, while it is still difficult to launch a project in docker, as there is an error, I will solve it in the near future! So for now, let's run the project using a virtual environment.**
Let's assume that python3 is installed on your local computer.
In the `ManagementCompanyWebServiceDjango/` directory, create a virtual environment by entering the commands:
```
python3 -m venv venv
source .venv/bin/activate
```
Virtual environment activated!
You need to start the server from the directory:
```
ManagementCompanyWebServiceDjango/management_company_interaction_project/
```
To install the required libraries to run the project, write a command in cmd:
```
pip install -r requirements.txt
```
It is necessary to make migrations by writing commands in cmd:
```
python manage.py makemigrations
python manage.py migrate
```
Also, you need to create an application administrator by typing the command in cmd:
```
python manage.py createsuperuser
```
Now you can start the server using the command:
```
python manage.py runserver
```
Everything, the server is running. Now you can go to the url `127.0.0.1:8000` to go to the home page of the site.
____
# Homepage
You went to url `127.0.0.1:8000`.
If you are not logged in, then on the main page you will be offered to log in (log in or register a new organization) by clicking on the necessary button. If authorized, you will be prompted to create new request or view active requests (pending or in progress).
____
# Registration
You went to url `127.0.0.1:8000/accounts/register`.
Everything is simple here: we enter the login and password for the organization, then we enter information about the organization itself. If a field is filled in incorrectly, for example a phone number, a hint is displayed after pressing the button that you entered the phone number incorrectly. An example of telephone input is shown below the input field. If you have successfully registered, you will be redirected to the login page.
____
# Login
You went to url `127.0.0.1:8000/accounts/login` (or redirected).
To log in, you need to specify the correct username and password. If you make a mistake four times, then your IP address will be blocked for 60 seconds: after this time, the blocking is removed, and you can try to log in again. After you successfully log in, you will be redirected to the page for creating request.
____
# Create request
You went to url `127.0.0.1:8000/request/new` (or redirected).
After you successfully log in, you will be redirected to the page for creating request.
This is where you fill out the basic information about the request and upload an image. If you do not upload an image, upload any file, but not an image or an invalid image (there is a footnote at the bottom about valid images), then after clicking on the buttons, a warning is displayed that you have uploaded an invalid image (or even a text file for example). In case of successful creation of the request, you will be redirected to the chat page for the request (let us create request number 1, then url : `127.0.0.1:8000/request/1`)
# Create worker of management company
Here we need to stop a little and separately create a user (s) who will respond to requests in the chat. To do this, go to url `127.0.0.1:8000/admin`.
We go into the previously created administrator account. Next, add the user manually - you need to come up with a username and password for the manager yourself. Click save.
Next, he needs to set the role of the manager. To do this, next to the "selected groups" tab, click on the add button (green plus sign), then enter "manager" in the name field. Click save. Then click save again to add the manager's rights.
Next, we will deal with the chat page.
____
# Chat request
You went to url `127.0.0.1:8000/request/1` (or redirected).
*The user cannot view other people's chats - this is important!*
One client (the creator of the request) and many managers who can change the status of the request can communicate in the chat.
Customers have only a button for sending a text message, while the manager will display buttons on the page for changing the status of the request and sending system messages. Initially, the status of the request is "pending". The manager can immediately close this request.
____
# List requests
You went to url `127.0.0.1:8000/request/list`
Here you can view the list of open requests. By clicking on one of them, you will be taken to the chat for this request.
<file_sep>CLOSE_STATUS_MESSAGE = 'Ваша заявка закрыта'
WORK_STATUS_MESSAGE = 'Ваша заявка одобрена, к вам отправляется мастер, дальнейшие подробности вам напишу позже'<file_sep>from django.contrib.auth.models import User
from django.db import models
class OrganisationProfile(models.Model):
representative = models.OneToOneField(User, on_delete=models.CASCADE,
verbose_name='Представитель/руководитель организации')
name = models.CharField(max_length=100, verbose_name='Наименование организации')
address = models.CharField(max_length=100, verbose_name='Адрес организации')
phone_number = models.CharField(max_length=17,
verbose_name='Номер телефона для связи')
def __str__(self):
return self.name
| 58fd60174a94f0658ff0bc748c0a9e768234b643 | [
"HTML",
"JavaScript",
"Markdown",
"Python",
"Text",
"Dockerfile"
] | 27 | Python | andrewShtopor/ManagementCompanyWebServiceDjango | 6db20cccb11c243a907c01125c61fcdf5fc0c303 | 152db1f48e7a607b30108e3ed0ac0601572942cd |
refs/heads/master | <file_sep>using UnityEngine;
using UnityEngine.SceneManagement;
public class NextLevel : MonoBehaviour
{
[SerializeField] private string nextLevel;
private void OnTriggerEnter2D( Collider2D collision )
{
if ( collision.CompareTag( "Player" ) )
{
SceneManager.LoadScene( nextLevel );
}
}
}
<file_sep>using UnityEngine;
public class PlayerController : MonoBehaviour
{
private const int TurnSpeed = 120;
// Called once per frame just before any physics
// Add custom physics here
private void FixedUpdate()
{
var leftInput = Input.GetKey( "left" ) || Input.GetKey( "a" );
var rightInput = Input.GetKey( "right" ) || Input.GetKey( "d" );
if ( leftInput && rightInput )
{
return;
}
Vector3 point = new Vector3( 0, 0, 0 );
if ( leftInput )
{
Vector3 axis = new Vector3( 0, 0, 1 );
transform.RotateAround( point, axis, Time.deltaTime * TurnSpeed );
}
if ( rightInput )
{
Vector3 axis = new Vector3( 0, 0, -1 );
transform.RotateAround( point, axis, Time.deltaTime * TurnSpeed );
}
}
}
| 2877a7d218d614e8f4557b26fb6a2dd054b16237 | [
"C#"
] | 2 | C# | jake-small/spin | 1e7147077f42d38d7799cc02a3ce64da1c166b32 | 6da8e0307d241c7ac67d137df0cdc1516ac39f79 |
refs/heads/master | <repo_name>misterdavemeister/video_poker<file_sep>/js/cardgame.js
var deck, hand1, hand2, hand3, hand4, hand5, discards, betAmount = 1;
var gameStarted = false, dealDealt = false, gameOver = true, gameScore;
var hold1 = false, hold2 = false, hold3 = false, hold4 = false, hold5 = false;
function gameState(event) {
/******************************************************
* checking game state before requested event *
* event === requested event *
* if checked conditions fail, event is denied *
******************************************************/
var dbtn = document.getElementById('deal');
var bbtn = document.getElementById('betButton');
switch (event) {
case "newGame": // newgame and deal buttons enabled -- bet button disabled
if (gameOver) { // indicates that last game was completed / this is a fresh game
/**** change button and game states ****/
toggleHoldBtns(); // visible if not currently visible, never invisible at this point
gameStarted = true;
dealDealt = false;
gameOver = false;
dbtn.disabled = false;
bbtn.disabled = true;
/** end change button and game states **/
newGame();
} else { // indicates that the game was started midgame
if (confirm("You are forfeiting this round. Are you sure?")) newGame();
else return;
}
break;
case "finalDeal": //newgame and bet buttons enabled -- deal disabled
if (dealDealt || gameStarted === false) return;
else {
/**** change button and game states ****/
gameStarted = false;
dealDealt = true;
gameOver = true;
dbtn.disabled = true;
bbtn.disabled = false;
/** end change button and game states **/
finalDeal();
}
break;
case "changeBet":
if (gameOver) changeBet();
else return;
break;
}
}
function Score() {
this.score = getCache();
this.addScore = function(add) {
this.score += add;
};
this.getScore = function() {
return "Bank: $" + addCommas(this.score);
};
this.getCacheScore = function() {
return this.score;
}
}
function newScore() {
gameScore = new Score();
}
function newGame() {
gameScore.score -= betAmount;
document.getElementById("score").innerHTML = gameScore.getScore();
document.getElementById("bet").innerHTML = "Bet: $" + addCommas(betAmount);
var msg = document.getElementById("message");
msg.innerHTML = "New hand dealt";
hold1 = false;
hold2 = false;
hold3 = false;
hold4 = false;
hold5 = false;
resetButtons();
init();
shuffle();
deal();
display();
}
function init() {
deck = new Stack();
hand1 = new Stack();
hand2 = new Stack();
hand3 = new Stack();
hand4 = new Stack();
hand5 = new Stack();
discards = new Stack();
deck.makeDeck(1);
}
function shuffle() {
if (deck == null) return;
deck.shuffle(1);
}
function deal() {
if (deck == null) return;
hand1.addCard(deck.deal());
hand2.addCard(deck.deal());
hand3.addCard(deck.deal());
hand4.addCard(deck.deal());
hand5.addCard(deck.deal());
}
function discard(hand) {
if (deck == null) return;
discards.combine(hand);
}
function reset() {
var el;
if (deck == null) return;
discards.combine(hand1);
discards.combine(hand2);
discards.combine(hand3);
discards.combine(hand4);
discards.combine(hand5);
deck.combine(discards);
}
function display() {
var el, i;
el = document.getElementById("card1");
while (el.firstChild != null)
el.removeChild(el.firstChild);
for (var i = 0; i < hand1.cardCount(); i++) {
node = hand1.cards[i].createNode();
el.appendChild(node);
}
var el, i;
el = document.getElementById("card2");
while (el.firstChild != null)
el.removeChild(el.firstChild);
for (var i = 0; i < hand2.cardCount(); i++) {
node = hand2.cards[i].createNode();
el.appendChild(node);
}
var el, i;
el = document.getElementById("card3");
while (el.firstChild != null)
el.removeChild(el.firstChild);
for (var i = 0; i < hand3.cardCount(); i++) {
node = hand3.cards[i].createNode();
el.appendChild(node);
}
var el, i;
el = document.getElementById("card4");
while (el.firstChild != null)
el.removeChild(el.firstChild);
for (var i = 0; i < hand4.cardCount(); i++) {
node = hand4.cards[i].createNode();
el.appendChild(node);
}
var el, i;
el = document.getElementById("card5");
while (el.firstChild != null)
el.removeChild(el.firstChild);
for (var i = 0; i < hand5.cardCount(); i++) {
node = hand5.cards[i].createNode();
el.appendChild(node);
}
}
function hold(btn, card) {
if (gameStarted && !(dealDealt)) {
if (btn.value === "HOLD") {
btn.value = "HELD";
btn.style.color = "blue";
if (card !== undefined) holdCard(card);
} else {
btn.value = "HOLD";
btn.style.color = "black";
if (card !== undefined) holdCard(card);
}
}
}
function holdCard(card) {
switch (card) {
case 'hand1':
hold1 ? hold1 = false : hold1 = true;
break;
case 'hand2':
hold2 ? hold2 = false : hold2 = true;
break;
case 'hand3':
hold3 ? hold3 = false : hold3 = true;
break;
case 'hand4':
hold4 ? hold4 = false : hold4 = true;
break;
case 'hand5':
hold5 ? hold5 = false : hold5 = true;
break;
default:
break;
}
}
function finalDeal() { //------------- UI Deal button's function -------------//
toggleHoldBtns();
var handArr = [hand1, hand2, hand3, hand4, hand5];
for (var i = 0; i < handArr.length; i++) {
switch (i) {
case 0:
if (!(hold1)) {
discard(handArr[i]);
handArr[i].addCard(deck.deal());
}
break;
case 1:
if (!(hold2)) {
discard(handArr[i]);
handArr[i].addCard(deck.deal());
}
break;
case 2:
if (!(hold3)) {
discard(handArr[i]);
handArr[i].addCard(deck.deal());
}
break;
case 3:
if (!(hold4)) {
discard(handArr[i]);
handArr[i].addCard(deck.deal());
}
break;
case 4:
if (!(hold5)) {
discard(handArr[i]);
handArr[i].addCard(deck.deal());
}
break;
default:
break;
}
}
resetButtons();
display();
getScore();
reset();
}
function toggleHoldBtns() {
var btnArr = ['hold1', 'hold2', 'hold3', 'hold4', 'hold5'];
for (var i = 0; i < btnArr.length; i++) {
document.getElementById(btnArr[i]).style.display = ((document.getElementById(btnArr[i]).style.display === 'none') || (document.getElementById(btnArr[i]).style.display === '')) ? 'inline' : 'none';
}
}
function resetButtons() {
var btnArr = ['hold1', 'hold2', 'hold3', 'hold4', 'hold5'];
for (var i = 0; i < btnArr.length; i++) {
if (document.getElementById(btnArr[i]).value === "HELD")
hold(document.getElementById(btnArr[i]));
}
}
function keyEvent(e) {
var key = e.keyCode;
switch (key) {
case 49:
hold(document.getElementById("hold1"), "hand1");
break;
case 50:
hold(document.getElementById("hold2"), "hand2");
break;
case 51:
hold(document.getElementById("hold3"), "hand3");
break;
case 52:
hold(document.getElementById("hold4"), "hand4");
break;
case 53:
hold(document.getElementById("hold5"), "hand5");
break;
case 66:
gameState('changeBet');
break;
case 67:
gameState('changeBet');
break;
case 13:
gameState('finalDeal');
break;
case 68:
gameState('finalDeal');
break;
case 32:
gameState('newGame');
break;
case 72:
gameState('newGame');
break;
case 78:
gameState('newGame');
break;
default:
break;
}
}
/************************* Determine Winning Hand & Score *************************/
function getScore() {
var h1R = hand1.cards[0].getRank();
var h2R = hand2.cards[0].getRank();
var h3R = hand3.cards[0].getRank();
var h4R = hand4.cards[0].getRank();
var h5R = hand5.cards[0].getRank();
var h1S = hand1.cards[0].getSuit();
var h2S = hand2.cards[0].getSuit();
var h3S = hand3.cards[0].getSuit();
var h4S = hand4.cards[0].getSuit();
var h5S = hand5.cards[0].getSuit();
var rankArr = [h1R, h2R, h3R, h4R, h5R];
var suitArr = [h1S, h2S, h3S, h4S, h5S];
var score = winningHands(rankArr, suitArr);
postScore(score);
}
function postScore(s) {
var scr = document.getElementById("score");
var msg = document.getElementById("message");
s *= betAmount;
gameScore.addScore(s);
scr.innerHTML = gameScore.getScore();
if (s > 0){
msg.innerHTML += " You won $" + addCommas(s);
animateMsg(msg);
}
cacheScore();
reset();
}
function animateMsg(el) {
//TODO: ANIMATE IN A CELEBRATORY FASHION BECAUSE GOOD THINGS HAPPENED
return;
}
function winningHands(ranks, suits) {
var msg = document.getElementById("message");
var r = convertRanks(ranks);
if (checkRoyalFlush(r, suits) === true) {
msg.innerHTML = "You got a royal flush!";
return 1000;
}
else if (checkStraightFlush(r, suits) === true) {
msg.innerHTML = "You got a straight flush!";
return 300;
}
else if (checkStraightFlushLow(r, suits) === true) {
msg.innerHTML = "You got a straight flush!";
return 300;
}
else if (checkf_o_a_k(r, suits) === true) {
msg.innerHTML = "You got a four of a kind!";
return 50;
}
else if (checkFullHouse(r, suits) === true) {
msg.innerHTML = "You got a full house!";
return 30;
}
else if (checkFlush(r, suits) === true) {
msg.innerHTML = "You got a flush!";
return 20;
}
else if (checkStraight(r, suits) === true) {
msg.innerHTML = 'You got a straight!';
return 15;
}
else if (checkStraightLow(r, suits) === true) {
msg.innerHTML = 'You got a straight!';
return 15;
}
else if (checkt_o_a_k(r, suits) === true) {
msg.innerHTML = "You got a three of a kind!";
return 10;
}
else if (checkTwoPair(r, suits) === true) {
msg.innerHTML = "You got a two pair!";
return 5;
}
else if (checkJHPair(r, suits) === true) {
msg.innerHTML = "You got jacks or better!";
return 2;
}
else {
msg.innerHTML = "You did not have a winning hand.";
return 0;
}
}
function convertRanks(ranks) {
var newRanks = ranks.map(function(rank) {
if (rank === "J") return 11;
else if (rank === "Q") return 12;
else if (rank === "K") return 13;
else if (rank === "A") return 14;
else return Number(rank);
});
return newRanks;
}
function convertRanksLow(ranks) {
var newRanks = ranks.map(function(rank) {
if (rank === 14) return 1;
else return Number(rank);
});
return newRanks;
}
function rankCounter(rankArray) {
var rSorted = rankArray.sort(function(a, b) {return a-b;});
var counter = 1;
var highestCount = 1;
for (var i = 1; i < rSorted.length; i++) {
if (rSorted[i] === rSorted[i - 1])
counter++;
if (counter > highestCount) highestCount = counter;
else
counter = 1;
}
return highestCount;
}
function resetCacheScore() {
var scr = document.getElementById("score");
if (confirm("Reset Score?")) {
localStorage['myScore'] = 25;
gameScore.score = 25;
scr.innerHTML = "Bank: $" + addCommas(gameScore.score);
}
}
function cacheScore() {
localStorage['myScore'] = gameScore.getCacheScore();
}
function getCache() {
var cachedScore = localStorage['myScore'] || 25;
return cachedScore;
}
function changeBet() {
var blabel = document.getElementById('bet');
var slabel = document.getElementById('score');
var msgAmount = "You have $" + addCommas(gameScore.getCacheScore()) + "\n\n Enter new amount below (numbers only)";
var amount = Number(prompt(msgAmount, betAmount));
if (amount > 0 || amount < 9999999999999990) {
betAmount = amount;
}
else betAmount = betAmount;
blabel.innerHTML = "Bet: $" + addCommas(betAmount);
slabel.innerHTML = gameScore.getScore();
}
function addCommas(num) {
if (num > 999999999999999999999) return num;
var numArr = String(num).split('').reverse();
var tmpStr = [];
if (Number(num) > 0) {
for (var i = 0; i < numArr.length; i++) {
if (i % 3 === 0 && i !== 0)
tmpStr.unshift(numArr[i] + ",");
else
tmpStr.unshift(numArr[i]);
}
return tmpStr.join('');
}
else {
tmpStr.push(numArr[0].shift);
for (var i = 0; i < numArr.length; i++) {
if (i % 3 === 0 && i !== 0)
tmpStr.unshift(numArr[i] + ",");
else
tmpStr.unshift(numArr[i]);
}
return tmpStr.join('');
}
}
/************************* Winning Hand Types *************************/
function checkRoyalFlush(r, s) {
var ts = s[0];
var t = false, j = false, q = false, k = false, a = false;
var flush = s.every(function(suit) {return ts === suit;});
r.forEach(function(rank) {
if (rank === 10) t = true;
if (rank === 11) j = true;
if (rank === 12) q = true;
if (rank === 13) k = true;
if (rank === 14) a = true;
});
if (flush && t && j && q && k && a) return true;
else return false;
}
function checkStraightFlush(r, s) {
var ts = s[0];
var rsorted = r.sort(function(a,b) {return a-b;});
var flush = s.every(function(suit) {return ts === suit;});
for (var i = 1; i < rsorted.length; i++) {
if ((rsorted[i] - 1) !== (rsorted[i - 1])) return false;
}
if (flush) return true;
else return false;
}
function checkStraightFlushLow(r, s) {
var nR = convertRanksLow(r);
var ts = s[0];
var rsorted = nR.sort(function(a,b) {return a-b;});
var flush = s.every(function(suit) {return ts === suit;});
for (var i = 1; i < rsorted.length; i++) {
if ((rsorted[i] - 1) !== (rsorted[i - 1])) return false;
}
if (flush) return true;
else return false;
}
function checkf_o_a_k(r, s) {
if (rankCounter(r) === 4) return true;
else return false;
}
function checkFullHouse(r, s) {
var rSorted = r.sort(function(a, b) {return a-b;});
var counter = 1, secondCounter = false;
var highestCount = 1;
var secondHighestCount = 1;
for (var i = 1; i < rSorted.length; i++) {
if (rSorted[i] === rSorted[i - 1]) {
counter++;
if (secondCounter === false) {
if (counter > highestCount) {
highestCount = counter;
}
}
else {
if (counter > secondHighestCount) {
secondHighestCount = counter;
}
}
}
else {
counter = 1;
secondCounter = true;
}
}
if (highestCount === 3 && secondHighestCount === 2) return true;
else if (highestCount === 2 && secondHighestCount === 3) return true;
else return false;
}
function checkFlush(r, s) {
var ts = s[0];
var flush = s.every(function(suit) {return ts === suit;});
return flush;
}
function checkStraight(r, s) {
var rsorted = r.sort(function(a,b) {return a-b;});
for (var i = 1; i < rsorted.length; i++) {
if ((rsorted[i] - 1) !== (rsorted[i - 1])) return false;
}
return true;
}
function checkStraightLow(r, s) {
var nR = convertRanksLow(r);
var rsorted = nR.sort(function(a,b) {return a-b;});
for (var i = 1; i < rsorted.length; i++) {
if ((rsorted[i] - 1) !== (rsorted[i - 1])) return false;
}
return true;
}
function checkt_o_a_k(r, s) {
if (rankCounter(r) === 3) return true;
else return false;
}
function checkTwoPair(r, s) {
var rSorted = r.sort(function(a, b) {return a-b;});
var pairCounter = 0;
for (var i = 1; i < rSorted.length; i++) {
if (rSorted[i] === rSorted[i - 1])
pairCounter++;
}
return pairCounter === 2;
}
function checkJHPair(r, s) {
var j = 0, q = 0, k = 0, a = 0;
r.forEach(function(rank) {
if (rank === 11) j++;
if (rank === 12) q++;
if (rank === 13) k++;
if (rank === 14) a++;
});
if (j === 2 || q === 2 || k === 2 || a === 2) return true;
else return false;
} | 878e0429692ee4acc44ce1890292907ee4df0607 | [
"JavaScript"
] | 1 | JavaScript | misterdavemeister/video_poker | 2f079f664b923b949ac4473c9b55b98b9fbde58e | 95277df3ce8091d49ae4cf89edb2cf11fb8db364 |
refs/heads/main | <file_sep><?php
use Illuminate\Http\Request;
use lemonpatwari\Contact\Http\Controllers\ContactFormController;
Route::get('contact',[ContactFormController::class,'index'])->name('contact.index');
Route::post('contact',[ContactFormController::class,'store'])->name('contact.store');
<file_sep><?php
namespace lemonpatwari\Contact\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Queue\SerializesModels;
class ContactMailable extends Mailable
{
use Queueable, SerializesModels;
public $description;
public $email;
/**
* Create a new message instance.
*
* @return void
*/
public function __construct($description, $email)
{
$this->description = $description;
$this->email = $email;
}
/**
* Build the message.
*
* @return $this
*/
public function build()
{
return $this->markdown('contact::contact.email')->with([
'message' => $this->description,
'email' => $this->email
]);
}
}
<file_sep># contact-package
This will send email to admin and save contact query in database
<file_sep><?php
return [
'email' => '<EMAIL>'
];
<file_sep><?php
namespace lemonpatwari\Contact\Http\Controllers;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Mail;
use lemonpatwari\Contact\Mail\ContactMailable;
use lemonpatwari\Contact\Models\ContactForm;
class ContactFormController extends Controller
{
public function index()
{
return view('contact::contact.index');
}
public function store(Request $request)
{
Mail::to(config('contact.email'))->send(new ContactMailable($request->description, $request->email));
ContactForm::create($request->all());
return back();
}
}
| 755ac80d8a692f163c8c4f5b7763593f57ddfc5d | [
"Markdown",
"PHP"
] | 5 | PHP | lemonpatwari/contact-package | 0e73298297392218983252162a545df3b19d90ac | 3fdeb728b2722b2e6b978f15db9e09127e347323 |
refs/heads/master | <file_sep>import { Component, OnInit } from "@angular/core";
import { SettingsService } from "src/app/services/settings/settings.service";
import { Ajustes } from "./../../interfaces/interfaces";
@Component({
selector: "app-account-settings",
templateUrl: "./account-settings.component.html",
styleUrls: ["./account-settings.component.css"],
})
export class AccountSettingsComponent implements OnInit {
constructor(private settingsService: SettingsService) {}
ngOnInit() {
const ajustes = this.settingsService.getAjustes();
this.colocarCheck(ajustes.tema);
}
cambiarColor(tema: string, link: any) {
this.aplicarCheck(link);
this.settingsService.aplicarAjustes(tema);
}
aplicarCheck(link: any) {
const selectores: any = document.getElementsByClassName("selector");
for (const selector of selectores) {
selector.classList.remove("working");
}
link.classList.add("working");
}
colocarCheck(tema: string) {
const selectores: any = document.getElementsByClassName("selector");
for (const selector of selectores) {
if (selector.getAttribute("data-theme") === tema) {
selector.classList.add("working");
break;
}
}
}
}
<file_sep>import { Component, OnInit } from "@angular/core";
@Component({
selector: "app-promesas",
templateUrl: "./promesas.component.html",
styles: [],
})
export class PromesasComponent implements OnInit {
constructor() {
this.contar()
.then((val) => {
console.log("termino", val);
})
.catch((error) => {
console.log(error);
});
}
contar() {
let contador = 0;
return new Promise<boolean>((res, rej) => {
const intervalo = setInterval(() => {
console.log(contador++);
if (contador === 3) {
res(true);
clearInterval(intervalo);
}
}, 1000);
});
}
ngOnInit() {}
}
<file_sep>import { Component, OnInit } from '@angular/core';
import { UsuarioService } from './../../services/usuario/usuario.service';
import { Usuario } from 'src/app/models/usuario.model';
@Component({
selector: 'app-profile',
templateUrl: './profile.component.html',
styles: []
})
export class ProfileComponent implements OnInit {
usuario: Usuario;
imgTemp: File;
imgPreview;
constructor(private usuarioService: UsuarioService) { }
ngOnInit() {
this.usuario = this.usuarioService.usuario;
}
seleccionarImagen(file: File) {
if (!file) {
this.imgTemp = null;
return;
}
this.imgTemp = file;
let reader = new FileReader();
let urlImgTemp = reader.readAsDataURL(file);
reader.onloadend = () => {
this.imgPreview = reader.result;
};
}
cambiarImagen() {
this.usuarioService.cambiarImagen(this.imgTemp, 'usuarios', this.usuario._id);
}
actualizar() {
this.usuarioService.actualizarUsuario(this.usuario).subscribe((result) => {
});
}
}
<file_sep>import { Component, OnInit, OnDestroy } from "@angular/core";
import { Observable, interval, Subscription } from "rxjs";
import { retry, map, filter } from "rxjs/operators";
@Component({
selector: "app-rxjs",
templateUrl: "./rxjs.component.html",
styles: [],
})
export class RxjsComponent implements OnInit, OnDestroy {
subscription: Subscription;
constructor() {
this.subscription = this.retornarObs()
//.pipe(retry(2))
.pipe(
map((resultado) => {
return Number(resultado.valor);
}),
filter((valor: any, index: number) => {
if (valor % 2 === 1) {
return true;
}
return false;
})
)
.subscribe(
(num) => {
console.log(num);
},
(error) => {
console.log(error);
},
() => {
console.log("complete");
}
);
}
retornarObs(): Observable<any> {
let cont = 0;
return new Observable((observer) => {
const intervalo = setInterval(() => {
cont++;
const salida = { valor: cont };
observer.next(salida);
if (cont === 10) {
clearInterval(intervalo);
observer.complete();
}
//if (cont === 2) {
// clearInterval(intervalo);
// observer.error("auxilio");
// }
}, 1000);
});
}
ngOnInit() {}
ngOnDestroy() {
this.subscription.unsubscribe();
}
}
<file_sep>import { Injectable } from '@angular/core';
import { environment } from './../../../environments/environment.prod';
import { HttpClient } from '@angular/common/http';
import Swal from 'sweetalert2';
const URL = environment.url;
@Injectable({
providedIn: 'root'
})
export class SubirArchivoService {
constructor(private http: HttpClient) { }
subirArchivo(archivo: File, tipo: string, id: string) {
return new Promise((res, rej) => {
const formData = new FormData();
const xhr = new XMLHttpRequest();
formData.append('imagen', archivo, archivo.name);
xhr.onreadystatechange = () => {
if (xhr.readyState === 4) {
if (xhr.status === 200) {
Swal.fire({
text: 'Imagen cargada',
icon: 'success',
confirmButtonText: 'Ok'
});
res(JSON.parse(xhr.response));
}
else {
rej(xhr.response);
}
}
};
const url = `${URL}/upload/${tipo}/${id}`;
xhr.open('PUT', url, true);
xhr.send(formData);
});
}
}
<file_sep>import {
EventEmitter,
Component,
OnInit,
Output,
ViewChild,
ElementRef,
} from "@angular/core";
@Component({
selector: "app-incrementador",
templateUrl: "./incrementador.component.html",
styleUrls: ["./incrementador.component.css"],
})
export class IncrementadorComponent implements OnInit {
@ViewChild("progresoInput") progresoInput: ElementRef;
@Output() cambioValor: EventEmitter<number> = new EventEmitter<number>();
progreso: number = 50;
leyenda: string = "";
constructor() {}
ngOnInit() {}
onChange(newValue: number) {
if (newValue >= 100) {
newValue = 100;
}
if (!newValue || newValue <= 0) {
newValue = 0;
}
this.progreso = newValue;
this.progresoInput.nativeElement = this.progreso;
this.cambioValor.emit(this.progreso);
}
cambiarValor(valor) {
this.progreso = this.progreso + valor;
this.cambioValor.emit(this.progreso);
this.progresoInput.nativeElement.focus();
}
}
<file_sep>import { Pipe, PipeTransform } from '@angular/core';
import { environment } from './../../environments/environment.prod';
const URL = environment.url;
@Pipe({
name: 'imagen'
})
export class ImagenPipe implements PipeTransform {
transform(img: string, tipo: string = 'usuario'): any {
const imgURL = `${URL}/img`;
if(!img) {
return `${imgURL}/usuarios/NOIMAGEN`;
}
return `${imgURL}/${tipo}/${img}`;
}
}
<file_sep>import { Injectable } from '@angular/core';
import { Usuario } from 'src/app/models/usuario.model';
import { HttpClient } from '@angular/common/http';
import { environment } from './../../../environments/environment';
import { map } from 'rxjs/operators';
import Swal from 'sweetalert2';
import { Router } from '@angular/router';
import { SubirArchivoService } from './../subirArchivo/subir-archivo.service';
const URL = environment.url;
@Injectable({
providedIn: 'root'
})
export class UsuarioService {
usuario: Usuario;
token: string;
constructor(private http: HttpClient, private router: Router, private subirArchivoService: SubirArchivoService) {
this.cargarStorage();
}
estaLogueado() {
return this.token && this.token.length > 1;
}
cargarStorage() {
this.token = localStorage.getItem('token') || '';
this.usuario = JSON.parse(localStorage.getItem('usuario')) || null;
}
logout() {
this.token = '';
this.usuario = null;
localStorage.removeItem('token');
localStorage.removeItem('usuario');
this.router.navigate(['/login']);
}
login(usuario: Usuario, recuerdame: boolean) {
return this.http.post(`${URL}/login`, usuario)
.pipe(
map((result: any) => {
this.guardarStorage(result.id, result.token, result.usuario);
})
);
}
guardarStorage(id: string, token: string, usuario: Usuario) {
localStorage.setItem('id', id);
localStorage.setItem('token', token);
localStorage.setItem('usuario', JSON.stringify(usuario));
this.token = token;
this.usuario = usuario;
}
crearUsuario(usuario: Usuario) {
return this.http.post(`${URL}/usuario`, usuario)
.pipe(
map((resp: any) => {
Swal.fire({
text: 'usuario creado',
icon: 'success',
confirmButtonText: 'Ok'
});
return resp.usuario;
})
);
}
actualizarUsuario(usuario: Usuario) {
return this.http.put(`${URL}/usuario/${usuario._id}?token=${this.token}`, usuario)
.pipe(map((result: any) => {
this.guardarStorage(result.usuario._id, this.token, result.usuario);
Swal.fire({
text: 'usuario actualizado',
icon: 'success',
confirmButtonText: 'Ok'
});
}));
}
cambiarImagen(archivo: File, tipo: string, id: string) {
this.subirArchivoService.subirArchivo(archivo, tipo, id).then((res: any) => {
this.usuario.img = res.img;
})
.catch(error => {
console.log(error);
});
}
}
| 31d93bbc07ba3cee1029948ec90fa0f01ecfe505 | [
"TypeScript"
] | 8 | TypeScript | dmz866/adminpro | 01a8ce84cdae7f36705aaa5f28c02170378a7caa | 45263ac18428d3ef085bc03f5f0e4c2a5bc1cfdd |
refs/heads/master | <file_sep>update=4/7/2014 6:26:09 PM
version=1
last_client=eeschema
[general]
version=1
[pcbnew]
version=1
LastNetListRead=FireFlyLid v0.2.net
UseCmpFile=1
PadDrill=0.000000000000
PadDrillOvalY=0.000000000000
PadSizeH=5.080000000000
PadSizeV=5.080000000000
PcbTextSizeV=1.500000000000
PcbTextSizeH=1.500000000000
PcbTextThickness=0.300000000000
ModuleTextSizeV=1.000000000000
ModuleTextSizeH=1.000000000000
ModuleTextSizeThickness=0.150000000000
SolderMaskClearance=0.000000000000
SolderMaskMinWidth=0.000000000000
DrawSegmentWidth=0.200000000000
BoardOutlineThickness=0.100000000000
ModuleOutlineThickness=0.150000000000
[pcbnew/libraries]
LibName1=battery_holders
LibName2=Board
LibName3=capacitors
LibName4=conn_9159
LibName5=conn_av
LibName6=conn_df12
LibName7=conn_d-sub
LibName8=conn_kk100
LibName9=conn_misc
LibName10=conn_modu
LibName11=conn_pc
LibName12=conn_strip
LibName13=crystal
LibName14=details
LibName15=dp_devices
LibName16=embedded_microcontrollers - Copy
LibName17=embedded_microcontrollers
LibName18=FireFly
LibName19=indicators
LibName20=misc_comp
LibName21=pin_strip
LibName22=pth_circuits
LibName23=pth_diodes
LibName24=pth_resistors
LibName25=relay
LibName26=smd_bga
LibName27=smd_cap
LibName28=smd_dil
LibName29=smd_diode
LibName30=smd_inductors
LibName31=smd_leds
LibName32=smd_lqfp
LibName33=smd_plcc
LibName34=smd_qfn
LibName35=smd_resistors
LibName36=smd_strip
LibName37=smd_trans
LibName38=switch
LibName39=to
LibDir=
[cvpcb]
version=1
NetIExt=net
[cvpcb/libraries]
EquName1=devcms
[eeschema]
version=1
LibDir=
NetFmtName=
RptD_X=0
RptD_Y=100
RptLab=1
LabSize=60
[eeschema/libraries]
LibName1=analog
LibName2=connectors
LibName3=device
LibName4=dp_devices
LibName5=embedded-microcontrollers
LibName6=logic
LibName7=memory
LibName8=microcontrollers
LibName9=MSP430G2553IPW28
LibName10=opto
LibName11=power
LibName12=relay
LibName13=rtx
LibName14=transistor
LibName15=vacuum
<file_sep>//***************************************************************************************
// <NAME>
// Nov 2013
// Built with Code Composer Studio v5
//***************************************************************************************
#ifndef SYSTEM_H_
#define SYSTEM_H_
#define FLOATING_ADC_FOR_RAND INCH_10 //temp sensor
void System_InitializeHW(void);
int System_Random(void);
#endif /* SYSTEM_H_ */
<file_sep>/*
* CapTouchLib.h
*
* Created on: Dec 15, 2013
* Author: ataft
*/
#ifndef CAPTOUCHLIB_H_
#define CAPTOUCHLIB_H_
typedef void ( * PFN_CAP_TOUCH_LIB_EVENT_SINK_VOID_VOID)(void);
void CapTouchLib_InitializeHW(void);
void CapTouchLib_InitializeApp(void);
void CapTouchLib_Tick(void);
//resets current button read.
//returns 1 when successfuly able to start button is sequence. If 1 then try again next tick
uint8_t CapTouchLib_EnableSmallButton(void);
void CapTouchLib_DisableSmallButton(void);
void CapTouchLib_Disable(void);
void CapTouchLib_Enable(void);
void CapTouchLib_RegisterLargeButtonPressSink(PFN_CAP_TOUCH_LIB_EVENT_SINK_VOID_VOID pfnEventSink);
void CapTouchLib_RegisterSmallButtonPressSink(PFN_CAP_TOUCH_LIB_EVENT_SINK_VOID_VOID pfnEventSink);
void CapTouchLib_RegisterLargeButtonReleaseSink(PFN_CAP_TOUCH_LIB_EVENT_SINK_VOID_VOID pfnEventSink);
void CapTouchLib_RegisterSmallButtonReleaseSink(PFN_CAP_TOUCH_LIB_EVENT_SINK_VOID_VOID pfnEventSink);
#endif /*CAPTOUCHLIB_H_ */
<file_sep>//***************************************************************************************
// <NAME>
// Nov 2013
// Built with Code Composer Studio v5
//***************************************************************************************
//TODO: large button controls on off
//TODO: refactor Play Song into multiple classes. One always one, One on when dark
//TODO: small button switches play states
//TODO: add storage of current play state to infomem on state change so restart enters correct one
//TODO: check that PWM is off and timer is off when main type is off
//TODO: add ability to change main WDT state calling times when system off and when in song that does not require a new open
//TODO: how to record light threshold? what UI?
//TODO: create new song that syncros
//TODO: new song types?
#include <msp430.h>
#include <stdint.h>
#include "MyTypes.h"
#include "system.h"
#include "SongRandom.h"
#include "CapTouchLib.h"
PFN_STATE_VOID_VOID g_pfnCurrentState;
//0 = false
uint8_t mSystemOn = 1;
uint8_t mPlayMode = 1;
void InitializeHW( void );
void InitializeApps( void );
void State_Default(void);
void LargeButtonPressed(void);
void LargeButtonReleased(void);
void SmallButtonPressed(void);
void SmallButtonReleased(void);
void OnDisableButtons(void);
void OnEnableButtons(void);
static const SPlaySongInfo mMain_tPlaySongInfo =
{
&g_pfnCurrentState,
OnDisableButtons,
OnEnableButtons
};
void main(void) {
WDTCTL = WDTPW + WDTHOLD; // Stop watchdog timer
g_pfnCurrentState = State_Default;
InitializeHW();
InitializeApps();
//set watchdog on timer move to somewhere else
WDTCTL = WDT_ADLY_16;
IFG1 &= ~(WDTIFG);
IE1 |= (WDTIE);
__enable_interrupt();
//call turn on
PlaySong_Entry(&mMain_tPlaySongInfo);
CapTouchLib_RegisterLargeButtonPressSink(LargeButtonPressed);
CapTouchLib_RegisterLargeButtonReleaseSink(LargeButtonReleased);
while(1)
{
__low_power_mode_0();
CapTouchLib_Tick();
g_pfnCurrentState();
}
}
void InitializeHW(void)
{
System_InitializeHW();
PlaySong_InitializeHW();
CapTouchLib_InitializeHW();
}
void InitializeApps(void)
{
PlaySong_InitializeApps();
CapTouchLib_InitializeApp();
}
void State_Default(void)
{
}
void LargeButtonPressed(void)
{
//turn off all songs not full off
//TODO: this will be replaced by a function on Main not playsong
PlaySong_Stop();
//turn on some leds
//TODO: put this in a function
P2OUT |= BIT4;
P3OUT |= BIT0;
P3OUT |= BIT2;
}
void LargeButtonReleased(void)
{
//Turn off leds
//TODO: put this in a function like the above button press
P2OUT &= ~(BIT4);
P3OUT &= ~(BIT0+BIT2);
//update system on/off and then respond
mSystemOn = ~mSystemOn;
if(mSystemOn)
{
//turn stuff on
}
else
{
//add stuff here to turn off further if needed
}
}
void OnDisableButtons(void)
{
CapTouchLib_Disable();
}
void OnEnableButtons(void)
{
CapTouchLib_Enable();
}
// Watchdog Timer interrupt service routine
#pragma vector=WDT_VECTOR
__interrupt void watchdog_timer(void)
{
//uint8_t tmp = __get_SR_register_on_exit();
//if(!(tmp & CPUOFF))
// mTimerMissed = 1;
__low_power_mode_off_on_exit();
}
<file_sep>MSP430_FireFlyJar
=================
MSP430 ValueLine FireFlyJar. With Max44009 I2C LUX Sensor, and CapTouch Buttons
<file_sep>//***************************************************************************************
// <NAME>
// Nov 2013
// Built with Code Composer Studio v5
//***************************************************************************************
#include <msp430.h>
#include <stdint.h>
#include <stdlib.h>
#include "System.h"
int adcGenRand8(void);
void System_InitializeHW(void)
{
//set clock
DCOCTL = 0; // Run at 16 MHz
BCSCTL1 = CALBC1_16MHZ; //
DCOCTL = CALDCO_16MHZ; //
BCSCTL3 |= LFXT1S1; // Set ACLK = Internal Osc
//set up all the ports
P1OUT = 0x00;
P1SEL = (BIT6)+(BIT7);
P1SEL2 = (BIT6)+(BIT7);
P1DIR = 0xFF; //p1.1 is captouch 1(large button). p1.6,p1.7 is used by I2C data and clk
//p2.3 as cap touch (enabled by Lib)
P2OUT = 0x00;
P2SEL = 0x00;
P2SEL2 = 0x00;
P2DIR = 0xFF;
//p3.7 as in for I2C Interrupt
P3OUT = 0x00;
P3SEL = 0x00;
P3SEL2 = 0x00;
P3DIR = 0x7F;
//initialize random num gen
srand(adcGenRand8());
}
int System_Random()
{
return rand();
}
int adcGenRand8(){
char bit;
unsigned char random;
for(bit = 0; bit < 8; bit++){
ADC10CTL1 |= FLOATING_ADC_FOR_RAND;
ADC10CTL0 |= SREF_1 + ADC10SHT_1 + REFON + ADC10ON;
ADC10CTL0 |= ENC + ADC10SC;
while(ADC10CTL1 & ADC10BUSY);
random <<= 1;
random |= (ADC10MEM & 0x01);
}
return random;
}
<file_sep>################################################################################
# Automatically-generated file. Do not edit!
################################################################################
# Each subdirectory must supply rules for building sources it contributes
Library/CTS_HAL.obj: ../Library/CTS_HAL.c $(GEN_OPTS) $(GEN_SRCS)
@echo 'Building file: $<'
@echo 'Invoking: MSP430 Compiler'
"C:/ti/ccsv5/tools/compiler/msp430/bin/cl430" -vmsp --abi=coffabi -g --include_path="C:/ti/ccsv5/ccs_base/msp430/include" --include_path="C:/ti/ccsv5/tools/compiler/msp430/include" --include_path="C:/Users/ataft/git/MSP430_FireFlyJar/FireFlyJar/Library" --include_path="C:/Users/ataft/git/MSP430_FireFlyJar/FireFlyJar/Application" --gcc --define=__MSP430G2553__ --diag_warning=225 --display_error_number --printf_support=minimal --preproc_with_compile --preproc_dependency="Library/CTS_HAL.pp" --obj_directory="Library" $(GEN_OPTS__FLAG) "$<"
@echo 'Finished building: $<'
@echo ' '
Library/CTS_Layer.obj: ../Library/CTS_Layer.c $(GEN_OPTS) $(GEN_SRCS)
@echo 'Building file: $<'
@echo 'Invoking: MSP430 Compiler'
"C:/ti/ccsv5/tools/compiler/msp430/bin/cl430" -vmsp --abi=coffabi -g --include_path="C:/ti/ccsv5/ccs_base/msp430/include" --include_path="C:/ti/ccsv5/tools/compiler/msp430/include" --include_path="C:/Users/ataft/git/MSP430_FireFlyJar/FireFlyJar/Library" --include_path="C:/Users/ataft/git/MSP430_FireFlyJar/FireFlyJar/Application" --gcc --define=__MSP430G2553__ --diag_warning=225 --display_error_number --printf_support=minimal --preproc_with_compile --preproc_dependency="Library/CTS_Layer.pp" --obj_directory="Library" $(GEN_OPTS__FLAG) "$<"
@echo 'Finished building: $<'
@echo ' '
<file_sep>/*
* I2CMaster.h
*/
#ifndef I2C_MASTER_H_
#define I2C_MASTER_H_
#define INTERSIL
#ifdef MAXIM
// Device addresses, right justified
#define DEVICE_SLV_ADDR 0x4A
#define INT_STATUS_REG 0x00
#define INT_ENABLE_REG 0x01
#define CONFIGURATION_REG 0x02
#define LUX_HIGH_REG 0x03
#define LUX_LOW_REG 0x04
#define HIGH_THRESH_REG 0x05
#define LOW_THRESH_REG 0x06
#define THRESH_TIMER_REG 0x07
#define THRESH_FULL_LUX 0xFF
#define THRESH_40_LUX 0x37
#define THRESH_30_LUX 0x35
#define THRESH_20_LUX 0x27
#define THRESH_10_LUX 0x17
#define THRESH_6_48_LUX 0x09
#define THRESH_5_76_LUX 0x08
#define THRESH_5_LUX 0x07
#define THRESH_4_32_LUX 0x06
#define THRESH_3_60_LUX 0x05
#define THRESH_2_88_LUX 0x04
#define THRESH_2_16_LUX 0x03
#define THRESH_1_44_LUX 0x02
#define THRESH_0_72_LUX 0x01
#define THRESH_0_LUX 0x00
#endif
#ifdef INTERSIL
#define DEVICE_SLV_ADDR 0x44 //or 0x88
#define COMMANDI_REG 0x00
#define COMMANDII_REG 0x01
#define DATA_LSB_REG 0x02
#define DATA_MSB_REG 0x03
#define LOW_THRESH_LSB_REG 0x04
#define LOW_THRESH_MSB_REG 0x05
#define HIGH_THRESH_LSB_REG 0x04
#define HIGH_THRESH_MSB_REG 0x05
#define COMMAND_ALS_CONTINUOUS 0xA0
#define COMMAND_POW_DWN 0x00
#define THRESH_FULL_LUX 0xFF
#define THRESH_10_LUX 0x17
#define THRESH_0_LUX 0x00
#endif
void I2C_Init( void );
uint8_t I2C_Device_SetTreshhold(uint8_t lowThreshhold, uint8_t highThreshhold);
double I2C_Device_GetLux(void);
uint8_t I2C_Device_GetLuxHighByte(void);
#endif /* I2C_MASTER_H_ */
<file_sep>/*
* BITHandlers.h
*
* Created on: Nov 18, 2013
* Author: ataft
*/
#ifndef MY_TYPES_H_
#define MY_TYPES_H_
#define bit_get(p,m) ((p) & (m))
#define bit_set(p,m) ((p) |= (m))
#define bit_clear(p,m) ((p) &= ~(m))
#define bit_flip(p,m) ((p) ^= (m))
#define bit_write(c,p,m) (c ? bit_set(p,m) : bit_clear(p,m))
#define BIT(x) (0x01 << (x))
typedef void ( * PFN_STATE_VOID_VOID ) (void);
#endif /* MY_TYPES_H_ */
<file_sep>//***************************************************************************************
// <NAME>
// Nov 2013
// Built with Code Composer Studio v5
//***************************************************************************************
#include <msp430.h>
#include <stdint.h>
#include <stdlib.h>
#include "MyTypes.h"
#include "SongRandom.h"
#include "BCM.h"
#include "I2CMaster.h"
static SPlaySongInfo const * mPlaySong_ptMainAppInfo;
//static PFN_STATE_VOID_VOID * mPlaySong_ppfnHostState;
//static PFN_STATE_VOID_VOID mPlaySong_ppfnPlayMode;
typedef struct {
int16_t FlashPoint;
int16_t FlashReset;
} PlaySong_FireflyMem;
typedef struct {
uint8_t Port;
uint8_t Pin;
} PlaySong_FireflyConst;
const uint8_t mPlaySong_auiMalePulse[50] = { /* pulse based on y=t*exp(t^2) */
0,37,74,107,140,168,194,215,230,244,251,254,255,254,251,246,236,224,210,195,178,162,146,130,
114,100,86,75,63,53,45,37,30,25,20,15,12,10,8,6,4,3,2,2,2,1,1,1,1,0
};
const uint8_t mPlaySong_auiFemalePulse[41] = { /* pulse based on y=t*exp(t^2) */
0,4,7,11,14,17,19,22,23,24,25,25,26,25,25,25,24,22,21,20,18,16,15,13,11,10,9,8,
6,5,5,4,3,3,2,2,1,1,1,1,0
};
static uint8_t mPlaySong_auiPortLevel[3][8] = {{0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0}};
static PlaySong_FireflyMem mPlaySong_affFireflyMem[19];
static const PlaySong_FireflyConst mPlaySong_affFireflyConst[] = {
{PORT1,0},
{PORT1,2},
{PORT1,3},
{PORT1,4},
{PORT1,5},
{PORT2,0},
{PORT2,1},
{PORT2,2},
{PORT2,4},
{PORT2,5},
{PORT2,6},
{PORT2,7},
{PORT3,0},
{PORT3,1},
{PORT3,2},
{PORT3,3},
{PORT3,4},
{PORT3,5},
{PORT3,6},
};
//Main States
void PlaySong_State_WaitForDark(void);
void PlaySong_State_Playing_Synchronizing(void);
void PlaySong_State_Playing_RandomFlashers(void);
void PlaySong_State_Stopped(void);
void PlaySong_Enter_WaitForDark(void);
void PlaySong_Enter_Playing(void);
//PlayMode States
//void PlaySong_PlayMode_State_Off(void);
//void PlaySong_PlayMode_State_StayOn(void);
//void PlaySong_PlayMode_State_DarkOnly(void);
//Helpers
void PlaySong_Synchronizing_Initialize(void);
void PlaySong_RandomFlashers_Initialize(void);
void PlaySong_InitializeHW(void)
{
//set up the i2c light sensor
I2C_Init();
BCM_InitializeHW();
}
void PlaySong_InitializeApps(void)
{
BCM_InitializeApp();
}
void PlaySong_Entry(SPlaySongInfo const * ptPlaySongInfo)
{
mPlaySong_ptMainAppInfo = ptPlaySongInfo;
*(mPlaySong_ptMainAppInfo->ppfnHostState) = PlaySong_State_Stopped;
}
void PlaySong_Stop()
{
BCM_Stop();
*(mPlaySong_ptMainAppInfo->ppfnHostState) = PlaySong_State_Stopped;
}
//void PlaySong_SetPlayMode(uint8_t mode)
//{
// //change this to use enum
// switch(mode)
// {
//
// case 0:
// mPlaySong_ppfnPlayMode = PlaySong_PlayMode_State_Off;
// break;
//
// case 1:
// mPlaySong_ppfnPlayMode = PlaySong_PlayMode_State_StayOn;
// break;
//
// case 2:
// mPlaySong_ppfnPlayMode = PlaySong_PlayMode_State_DarkOnly;
// break;
//
// }
// mPlaySong_ppfnPlayMode();
//}
////PlayMode States
//void PlaySong_PlayMode_State_Off(void)
//{
// BCM_Stop();
// *(mPlaySong_ptMainAppInfo->ppfnHostState) = PlaySong_State_Stopped;
//}
//void PlaySong_PlayMode_State_StayOn(void)
//{
// PlaySong_Enter_Playing();
//}
//void PlaySong_PlayMode_State_DarkOnly(void)
//{
// PlaySong_Enter_WaitForDark();
//}
//Maint States
void PlaySong_State_Stopped(void)
{
//do nothing
}
void PlaySong_Enter_WaitForDark(void)
{
//stop the BCM
BCM_Stop();
//disable the buttons
//mPlaySong_ptMainAppInfo->pfnCallback_ButtonDisable();
//check current lux level. pull threshold value from somewhere else next
uint8_t curLux = 0;
curLux = I2C_Device_GetLuxHighByte();
if(THRESH_10_LUX < curLux)
{
I2C_Device_SetTreshhold(THRESH_10_LUX, THRESH_FULL_LUX);
//enable the p3.7 interrupts apparently there are no port 3 interupts doing active polling in next state
//P3IES |= (BIT7);
//P3IFG &= ~(BIT7);
//P3IE |= (BIT7);
//transition to new state
*(mPlaySong_ptMainAppInfo->ppfnHostState) = PlaySong_State_WaitForDark;
}
else
{
PlaySong_Enter_Playing();
}
//mPlaySong_ptMainAppInfo->pfnCallback_ButtonEnable();
}
void PlaySong_State_WaitForDark(void)
{
//check 3.7 for low
if((P3IN & BIT7) == 0)
{
PlaySong_Enter_Playing();
return;
}
}
void PlaySong_Enter_Playing(void)
{
//mPlaySong_ptMainAppInfo->pfnCallback_ButtonDisable();
// if(*mPlaySong_ppfnPlayMode == PlaySong_PlayMode_State_DarkOnly)
// {
// //set the on light alert
// //pull this value from somewhere else next
// I2C_Device_SetTreshhold(THRESH_0_LUX, THRESH_10_LUX);
//
// //enable the p3.7 interrupts no interupts will have to poll on each play in next step
// //P3IES |= (BIT7);
// //P3IFG &= ~(BIT7);
// //P3IE |= (BIT7);
// }
//init current song
PlaySong_RandomFlashers_Initialize();
BCM_EncodeTimeSliceAllPins(PORT1, mPlaySong_auiPortLevel[PORT1]);
BCM_EncodeTimeSliceAllPins(PORT2, mPlaySong_auiPortLevel[PORT2]);
BCM_EncodeTimeSliceAllPins(PORT3, mPlaySong_auiPortLevel[PORT3]);
//mPlaySong_ptMainAppInfo->pfnCallback_ButtonEnable();
//transition to new state
*(mPlaySong_ptMainAppInfo->ppfnHostState) = PlaySong_State_Playing_RandomFlashers;
}
void PlaySong_State_Playing_Synchronizing(void)
{
}
void PlaySong_Synchronizing_Initialize(void)
{
}
void PlaySong_State_Playing_RandomFlashers(void)
{
//check 3.7 for low
if((P3IN & BIT7) == 0)
{
PlaySong_Enter_WaitForDark();
return;
}
uint8_t i;
for(i = 0; i < 19; i++)
{
if(mPlaySong_affFireflyMem[i].FlashPoint++ < 0)
{
continue;
}
if(mPlaySong_affFireflyMem[i].FlashPoint >= 0)
{
mPlaySong_auiPortLevel[mPlaySong_affFireflyConst[i].Port][mPlaySong_affFireflyConst[i].Pin] = mPlaySong_auiMalePulse[mPlaySong_affFireflyMem[i].FlashPoint];
}
if(49 == mPlaySong_affFireflyMem[i].FlashPoint)
{
mPlaySong_affFireflyMem[i].FlashPoint = mPlaySong_affFireflyMem[i].FlashReset;
}
}
BCM_EncodeTimeSliceAllPins(PORT1, mPlaySong_auiPortLevel[PORT1]);
BCM_EncodeTimeSliceAllPins(PORT2, mPlaySong_auiPortLevel[PORT2]);
BCM_EncodeTimeSliceAllPins(PORT3, mPlaySong_auiPortLevel[PORT3]);
}
#define BASE_RANDOM_FLASH_PERIOD -600
void PlaySong_RandomFlashers_Initialize(void)
{
uint8_t i;
for(i = 0; i < 19; i++)
{
//set the period for this fly
mPlaySong_affFireflyMem[i].FlashReset = BASE_RANDOM_FLASH_PERIOD - rand() % 100;
//set the start point for this fly
mPlaySong_affFireflyMem[i].FlashPoint = 0-(rand() % mPlaySong_affFireflyMem[i].FlashReset);
//clear the pin for this fly
mPlaySong_auiPortLevel[mPlaySong_affFireflyConst[i].Port][mPlaySong_affFireflyConst[i].Pin] = 0;
}
}
// Port 3 interrupt service routine apparently there is no interupt on port 3
/*
#pragma vector=PORT3_VECTOR
__interrupt void Port_3(void)
{
//check if p2.7 was triggered
if(P3IE & BIT7)
{
if(P3IFG & BIT7)
{
P3IE &= ~(BIT7); // P3.7 Interrupt disabled
P3IFG &= ~(BIT7); // P3.7 IFG cleared
if(*mPlaySong_ppfnHostState == PlaySong_State_WaitForDark)
{
PlaySong_Enter_Playing();
return;
}
if(*mPlaySong_ppfnHostState == PlaySong_State_Playing_Synchronizing)
{
PlaySong_Enter_WaitForDark();
return;
}
if(*mPlaySong_ppfnHostState == PlaySong_State_Playing_RandomFlashers)
{
PlaySong_Enter_WaitForDark();
return;
}
}
}
}
*/
<file_sep>################################################################################
# Automatically-generated file. Do not edit!
################################################################################
# Add inputs and outputs from these tool invocations to the build variables
C_SRCS += \
../CapTouch/CTS_HAL.c \
../CapTouch/CTS_Layer.c \
../CapTouch/CapTouchLib.c \
../CapTouch/structure.c
OBJS += \
./CapTouch/CTS_HAL.obj \
./CapTouch/CTS_Layer.obj \
./CapTouch/CapTouchLib.obj \
./CapTouch/structure.obj
C_DEPS += \
./CapTouch/CTS_HAL.pp \
./CapTouch/CTS_Layer.pp \
./CapTouch/CapTouchLib.pp \
./CapTouch/structure.pp
C_DEPS__QUOTED += \
"CapTouch\CTS_HAL.pp" \
"CapTouch\CTS_Layer.pp" \
"CapTouch\CapTouchLib.pp" \
"CapTouch\structure.pp"
OBJS__QUOTED += \
"CapTouch\CTS_HAL.obj" \
"CapTouch\CTS_Layer.obj" \
"CapTouch\CapTouchLib.obj" \
"CapTouch\structure.obj"
C_SRCS__QUOTED += \
"../CapTouch/CTS_HAL.c" \
"../CapTouch/CTS_Layer.c" \
"../CapTouch/CapTouchLib.c" \
"../CapTouch/structure.c"
<file_sep>/*
* I2CMaster.c
*/
#include <msp430.h>
#include <stdint.h>
#include "I2CMaster.h"
static volatile uint16_t I2CNumBytes;
static volatile uint8_t Ack;
static volatile uint8_t * I2CRxBuffer;
static volatile uint8_t * I2CTxBuffer;
static volatile uint16_t I2CStop;
uint8_t I2C_Write( uint8_t , uint8_t * , uint16_t );
uint8_t I2C_Read( uint8_t , uint8_t * , uint16_t );
#ifdef MAXIM
uint8_t I2C_Device_SetTreshhold(uint8_t lowThreshhold, uint8_t highThreshold)
{
uint8_t writeVal[2];
//set up to go from light to dark
//turn off INT to clear value
writeVal[0] = INT_ENABLE_REG;
writeVal[1] = 0x00;
if (!I2C_Write(DEVICE_SLV_ADDR, writeVal, 2))
{
return 0;
}
writeVal[0] = LOW_THRESH_REG;
writeVal[1] = lowThreshhold;
if (!I2C_Write(DEVICE_SLV_ADDR, writeVal, 2))
{
return 0;
}
writeVal[0] = HIGH_THRESH_REG;
writeVal[1] = highThreshold;
if (! I2C_Write(DEVICE_SLV_ADDR, writeVal, 2))
{
return 0;
}
writeVal[0] = THRESH_TIMER_REG;
writeVal[1] = 0x05;
if (!I2C_Write(DEVICE_SLV_ADDR, writeVal, 2))
{
return 0;
}
writeVal[0] = INT_ENABLE_REG;
writeVal[1] = 0x01;
if (!I2C_Write(DEVICE_SLV_ADDR, writeVal, 2))
{
return 0;
}
return 1;
}
double I2C_Device_GetLux(void)
{
uint8_t high;
uint8_t low;
uint8_t writeVal[2];
uint8_t retVal;
//__enable_interrupt();
//read from MAXIM CHIP
writeVal[0] = INT_STATUS_REG;
//retVal = I2C_Write(DEVICE_SLV_ADDR, writeVal, 1);
//retVal = I2C_Read(DEVICE_SLV_ADDR, &val, 1);
//read lux value
writeVal[0] = LUX_HIGH_REG;
retVal = I2C_Write(DEVICE_SLV_ADDR, writeVal, 1);
retVal = I2C_Read(DEVICE_SLV_ADDR, &high, 1);
writeVal[0] = LUX_LOW_REG;
retVal = I2C_Write(DEVICE_SLV_ADDR, writeVal, 1);
retVal = I2C_Read(DEVICE_SLV_ADDR, &low, 1);
volatile uint8_t e = (high >> 4) & 0x0F;
volatile uint16_t lux = 1;
while(e>0)
{
lux *= 2;
e--;
}
volatile uint8_t m = high & 0x0F;
volatile double lowLux = lux * m * 0.72;
m = m << 4;
m = m | (0x0F & low);
volatile double highLux = lux * m * 0.045;
return highLux;
}
uint8_t I2C_Device_GetLuxHighByte(void)
{
uint8_t high;
uint8_t reg = LUX_HIGH_REG;
//read lux value
I2C_Write(DEVICE_SLV_ADDR, ®, 1);
I2C_Read(DEVICE_SLV_ADDR, &high, 1);
return high;
}
#endif
#ifdef INTERSIL
uint8_t I2C_Device_SetTreshhold(uint8_t lowThreshhold, uint8_t highThreshold)
{
return 0;
}
double I2C_Device_GetLux(void)
{
uint8_t writeVal[2];
//set up to go from light to dark
//turn off INT to clear value
writeVal[0] = COMMANDI_REG;
writeVal[1] = COMMAND_ALS_CONTINUOUS;
if (!I2C_Write(DEVICE_SLV_ADDR, writeVal, 2))
{
return 0;
}
uint8_t reg = DATA_LSB_REG;
//read lux value
I2C_Write(DEVICE_SLV_ADDR, ®, 1);
I2C_Read(DEVICE_SLV_ADDR, &writeVal, 2);
return 0;
}
uint8_t I2C_Device_GetLuxHighByte(void)
{
return 0;
}
#endif
void I2C_Init( void )
{
UCB0CTL1 |= UCSWRST; // Enable SW reset
UCB0CTL0 = UCMST + UCMODE_3 + UCSYNC; // 7-bit addressing, single-master environment, I2C Master, synchronous mode
UCB0CTL1 = UCSSEL_2 + UCSWRST; // Use SMCLK, keep SW reset
UCB0BR0 = 80; // fSCL = SMCLK/UCB0BR1
UCB0BR1 = 0;
UCB0I2CIE = UCNACKIE; // Enable not-acknowledge interrupt
UCB0CTL1 &= ~UCSWRST; // Clear SW reset, resume operation
IE2 |= UCB0TXIE + UCB0RXIE; // Enable TX&RX interrupts
}
uint8_t I2C_Write( uint8_t sladdr , uint8_t *data , uint16_t n )
{
//
Ack = 1; // Return value
//
I2CTxBuffer = data; // TX array start address
I2CNumBytes = n; // Update counter
UCB0I2CSA = sladdr; // Slave address (Right justified, bits6-0)
//
//uint16_t uiInterruptState = __get_SR_register();
//_enable_interrupts();
//
UCB0CTL1 |= UCTR + UCTXSTT; // Send I2C start condition, I2C TX mode
LPM0; // Enter LPM0
//
while( UCB0CTL1 & UCTXSTP ); // I2C stop condition sent?
//
//_bis_SR_register(uiInterruptState);
return Ack;
}
uint8_t I2C_Read( uint8_t sladdr , uint8_t *data , uint16_t n )
{
//
Ack = 1; // Return value
//
I2CRxBuffer = data; // Start of RX buffer
UCB0I2CSA = sladdr; // Slave address (Right justified, bits6-0)
//
UCB0CTL1 &= ~UCTR; // I2C RX mode
//
if( n == 1 )
{
I2CNumBytes = 0; // Update counter
//
//__disable_interrupt();
UCB0CTL1 |= UCTXSTT; // Send I2C start condition, I2C RX mode
while( UCB0CTL1 & UCTXSTT ); // I2C start condition sent?
UCB0CTL1 |= UCTXSTP; // Send I2C stop condition
I2CStop = 1; // I2C stop condition sent
//__enable_interrupt();
}
else if( n > 1 )
{
I2CStop = 0; // I2C stop condition not sent yet
I2CNumBytes = n - 2; // Update counter
UCB0CTL1 |= UCTXSTT; // Send I2C start condition
}
LPM0; // Enter LPM0
//
while( UCB0CTL1 & UCTXSTP ); // I2C stop condition sent?
//
return Ack;
}
#ifdef I2C_PING
uint16_t I2C_Ping( uint8_t sladdr )
{
//
UCB0I2CSA = sladdr; // Slave address (Right justified, bits6-0)
//
__disable_interrupt();
UCB0CTL1 |= UCTR + UCTXSTT + UCTXSTP; // I2C start condition, I2C TX mode, I2C stop condition
while( UCB0CTL1 & UCTXSTP ); // I2C stop condition sent?
Ack = !(UCB0STAT & UCNACKIFG); // I2C start condition akd'd or not?
__enable_interrupt();
//
return Ack;
}
#endif /* !I2C_PING */
#pragma vector = USCIAB0TX_VECTOR
__interrupt void USCIAB0TX_ISR(void)
{
if( IFG2 & UCB0RXIFG ) // RX mode
{
if( I2CNumBytes == 0 )
{
// I2CStop is used just to make sure that we leave LPM0 at the right time and not
// before
if( I2CStop )
{
_low_power_mode_off_on_exit( ); // Exit LPM0
}
else
{
UCB0CTL1 |= UCTXSTP; // I2C stop condition
I2CStop = 1; // I2C stop condition sent
}
}
else
{
I2CNumBytes--; // Decrement counter
}
*I2CRxBuffer++ = UCB0RXBUF; // Read RX data. This automatically clears UCB0RXIFG
}
else // TX mode
{
if( I2CNumBytes ) // Check counter
{
UCB0TXBUF = *I2CTxBuffer++; // Load TX buffer. This automatically clears UCB0TXIFG
I2CNumBytes--; // Decrement counter
}
else
{
UCB0CTL1 |= UCTXSTP; // I2C stop condition
IFG2 &= ~UCB0TXIFG; // Clear USCI_B0 TX int flag
_low_power_mode_off_on_exit( ); // Exit LPM0
}
}
}
#pragma vector = USCIAB0RX_VECTOR
__interrupt void USCIAB0RX_ISR(void)
{
// Not-acknowledge interrupt. This flag is set when an acknowledge is expected
// but is not received. UCNACKIFG is automatically cleared when a START condition
// is received.
if( UCB0STAT & UCNACKIFG )
{
UCB0CTL1 |= UCTXSTP; // I2C stop condition
Ack = 0; // Return value
UCB0STAT &= ~UCNACKIFG; // Clear interrupt flag
}
// Arbitration-lost. When UCALIFG is set the UCMST bit is cleared and the I2C
// controller becomes a slave. This can only happen in a multimaster environment
// Start condition detected interrupt. UCSTTIFG only used in slave mode.
// Stop condition detected interrupt. UCSTPIFG only used in slave mode.
_low_power_mode_off_on_exit( ); // Exit LPM0
}
<file_sep>//***************************************************************************************
// <NAME>
// Nov 2013
// Built with Code Composer Studio v5
//***************************************************************************************
#ifndef SONGRANDOM_H_
#define SONGRANDOM_H_
typedef void ( * PFN_PLAYSONG_CALLBACK_ON_EXIT_VOID_VOID )( void );
//example:
//void Main_OnExit(void);
void PlaySong_InitializeHW(void);
void PlaySong_InitializeApps(void);
void PlaySong_Entry(SPlaySongInfo const * ptPlaySongInfo);
void PlaySong_SetPlayMode(uint8_t);
void PlaySong_Stop();
#endif /* SONGRANDOM_H_ */
<file_sep>/*
* BCM.h
*
* Created on: Nov 10, 2013
* Author: ataft
*/
#ifndef BCM_H_
#define BCM_H_
#define BCM_RESOLUTION 8
#define BCM_TIMER_OFFSET 7 //shifting 8 positions for now but that gets us ~122 Hz. might try shifting 7(245Hz) or 6(490Hz)
#define PORT1 0
#define PORT2 1
#define PORT3 2
void BCM_InitializeHW(void);
void BCM_InitializeApp(void);
void BCM_Stop(void);
void BCM_EncodeTimeSliceAllPins(uint8_t port, uint8_t *);
#endif /* BCM_H_ */
<file_sep>/*
* BCM.c
*
* Created on: Nov 10, 2013
* Author: ataft
*/
#include <msp430.h>
#include <stdint.h>
#include "MyTypes.h"
#include "BCM.h"
//this is a 2 dimensional array, the first dimmension is the port number
//the second is the time slice in decending order with the 0 index = lsb and 7 = msb of each pwm value
static uint8_t mBCM_uiTimeSlices[3][BCM_RESOLUTION];
static volatile uint8_t mBCM_uiBitPosition = 0;
static PFN_STATE_VOID_VOID mBCM_pfnMode;
static union BCM_PinOn
{
uint32_t fullFlag;
uint8_t port[4];
} mBCM_uiPinOn;
void BCM_Mode_On( void );
void BCM_Mode_Off( void );
void BCM_UpdateMode(void);
void BCM_InitializeHW( void )
{
//set up the timer
TA1CTL = TASSEL_2 + ID_0; // Setup timer, SMCLK/1
}
void BCM_InitializeApp( void )
{
mBCM_uiPinOn.fullFlag = 0;
mBCM_pfnMode = BCM_Mode_Off;
}
void BCM_Stop(void)
{
mBCM_uiPinOn.fullFlag = 0;
mBCM_pfnMode = BCM_Mode_On;
BCM_UpdateMode();
}
void BCM_UpdateMode(void)
{
mBCM_pfnMode();
}
void BCM_Mode_On( void )
{
if(!mBCM_uiPinOn.fullFlag)
//no pins on so turn off
{
TA1CCTL1 &= ~CCIE; // Disable timer interrupt
TA1CTL &= ~((MC0)&(MC1)); //turn off timer
P1OUT = 0x00; //set all ports to 0
P2OUT = 0x00;
P3OUT = 0x00;
mBCM_pfnMode = BCM_Mode_Off;
}
//some pin on so do nothing
}
void BCM_Mode_Off( void )
{
if(mBCM_uiPinOn.fullFlag)
//some pin on so turn on
{
TA1CTL |= MC1; //continuous mode
TA1CTL &= ~(MC0); //continuous mode
TA1CCTL1 |= CCIE; // Enable timer interrupt
TA1CCR1 = 1 << BCM_TIMER_OFFSET; //set first stop
mBCM_pfnMode = BCM_Mode_On;
}
//all pins off so do nothing
}
//void BCM_ClearTimeSlice(gBCM_tPort port)
//{
// uint8_t i;
// //set all the time slices to 0
// for(i = 0; i<BCM_RESOLUTION; i++)
// {
// mBCM_uiTimeSlices[port][i] = 0x00;
// }
//}
//void BCM_EncodeTimeSlice(gBCM_tPort port, uint8_t pin, uint8_t value)
//{
// //loop through each timeslice value
// uint8_t i;
// for(i = BCM_RESOLUTION-1; i==0; i--)
// {
// //set the pin on or off in the timeslice
// (value & (1 << i)) ? (mBCM_uiTimeSlices[port][i] |= (1 << i)) : (mBCM_uiTimeSlices[port][i] &= ~(1 << i));
// }
//}
extern void BCM_BytePivot(uint8_t * src, uint8_t * dest, uint8_t * pinFlag);
void BCM_EncodeTimeSliceAllPins(uint8_t port, uint8_t * values)
{
//uint16_t isInterruptState = __get_SR_register();
//__disable_interrupt();
BCM_BytePivot(values, mBCM_uiTimeSlices[port], &mBCM_uiPinOn.port[port]);
mBCM_pfnMode();
//_bis_SR_register(isInterruptState);
// uint8_t portbits = 0;
// uint8_t bitvalue;
//
// uint8_t bitpos;
// for(bitpos = 0; bitpos < BCM_RESOLUTION; bitpos++)
// {
// portbits = 0;
// bitvalue = 1;
// uint8_t pin;
// for( pin = 0; pin < 8; pin++)
// {
// if(values[pin] & (1 << bitpos)) portbits |= bitvalue;
// bitvalue = bitvalue << 1;
// }
// mBCM_uiTimeSlices[port][bitpos] = portbits;
// }
}
//BCM interupt on TIMER1_A0
#pragma vector = TIMER1_A1_VECTOR
__interrupt void Timer1_A1 (void)
{
//clear interrupt flag
volatile unsigned x = TA1IV; // Clear interrupt flag
mBCM_uiBitPosition++;
mBCM_uiBitPosition &= 7; //keep values of 0-7
//update the port values
P1OUT = mBCM_uiTimeSlices[PORT1][mBCM_uiBitPosition];
P2OUT = mBCM_uiTimeSlices[PORT2][mBCM_uiBitPosition];
P3OUT = mBCM_uiTimeSlices[PORT3][mBCM_uiBitPosition];
//update the timer compare
TA1CCR1 = BIT(mBCM_uiBitPosition) << BCM_TIMER_OFFSET;
TA1CTL |= TACLR;
}
<file_sep>/*
* CapTouchLib.c
*
* Created on: Dec 15, 2013
* Author: ataft
*/
#include <msp430.h>
#include <stdint.h>
#include "MyTypes.h"
#include "CapTouchLib.h"
typedef struct StructButtonInfo
//defines the needs for a cap button
{
PFN_STATE_VOID_VOID pfnCurrentState;
struct StructButtonInfo* tNextButton;
PFN_CAP_TOUCH_LIB_EVENT_SINK_VOID_VOID pfnPressEventSink;
PFN_CAP_TOUCH_LIB_EVENT_SINK_VOID_VOID pfnReleaseEventSink;
uint16_t uiBaseSenseLevel;
uint8_t *uiPortSelAddress; //can be removed if both are on the same port
uint8_t *uiPortSel2Address; //can be removed if both are on the same port
uint8_t uiPin;
} SButtonInfo;
static SButtonInfo* mCapTouchLib_ptCurrentButton;
static SButtonInfo mCapTouchLib_tLargeButton;
static SButtonInfo mCapTouchLib_tSmallButton;
#define CAPTOUCHLIB_KEY_COMPARE 2500 // Defines the min count for a "key press"
// Set to ~ half the max delta expected
static uint16_t mCapTouchLib_uiMeasurementCount;
static uint16_t mCapTouchLib_uiDeltaCount;
static uint8_t mCapTouchLib_ubContextSaveSel;
static uint8_t mCapTouchLib_ubContextSaveSel2;
//static PFN_STATE_VOID_VOID mCapTouchLib_pfnCurrentState;
void CapTouchLib_DefaultEventSink(void);
//states
void CapTouchLib_State_InitButton(void);
void CapTouchLib_State_WaitForPress(void);
void CapTouchLib_State_PressDelay(void);
void CapTouchLib_State_WaitForRelease(void);
void CapTouchLib_State_ReleaseDelay(void);
void CapTouchLib_State_Disabled(void);
//Helpers
void CapTouchLib_EnterInitButton(void);
void CapTouchLib_EnterWaitForPress(void);
void CapTouchLib_EnterWaitForRelease(void);
void CapTouchLib_SetButtonForRead(void);
void CapTouchLib_ReadButtonValue(void);
void CapTouchLib_InitializeHW( void )
{
//set up the timer
TA0CTL = TASSEL_3 + ID_3 + MC_2; // TACLK, cont mode, Divide input by 8 to deal with slower wdt
TA0CCTL1 = CM_3+CCIS_2+CAP; // Pos&Neg,GND,Cap
//watchdog timer controlled externally
}
void CapTouchLib_InitializeApp( void )
{
//set defaults with just LargeButton on
mCapTouchLib_tLargeButton.uiPortSelAddress = (uint8_t *)&P1SEL;
mCapTouchLib_tLargeButton.uiPortSel2Address = (uint8_t *)&P1SEL2;
mCapTouchLib_tLargeButton.uiPin = BIT1; //pin 1
mCapTouchLib_tLargeButton.pfnPressEventSink = CapTouchLib_DefaultEventSink;
mCapTouchLib_tLargeButton.pfnReleaseEventSink = CapTouchLib_DefaultEventSink;
mCapTouchLib_tLargeButton.tNextButton = &mCapTouchLib_tLargeButton;
mCapTouchLib_tSmallButton.uiPortSelAddress = (uint8_t *)&P2SEL;
mCapTouchLib_tSmallButton.uiPortSel2Address = (uint8_t *)&P2SEL2;
mCapTouchLib_tSmallButton.uiPin = BIT3; //pin 3
mCapTouchLib_tSmallButton.pfnPressEventSink = CapTouchLib_DefaultEventSink;
mCapTouchLib_tSmallButton.pfnReleaseEventSink = CapTouchLib_DefaultEventSink;
mCapTouchLib_tSmallButton.tNextButton = &mCapTouchLib_tLargeButton;
mCapTouchLib_ptCurrentButton = &mCapTouchLib_tLargeButton;
CapTouchLib_EnterInitButton();
}
void CapTouchLib_Tick(void)
{
mCapTouchLib_ptCurrentButton->pfnCurrentState();
}
void CapTouchLib_RegisterLargeButtonPressSink(PFN_CAP_TOUCH_LIB_EVENT_SINK_VOID_VOID pfnEventSink)
{
if(0 == pfnEventSink)
{
mCapTouchLib_tLargeButton.pfnPressEventSink = CapTouchLib_DefaultEventSink;
}
else
{
mCapTouchLib_tLargeButton.pfnPressEventSink = pfnEventSink;
}
}
void CapTouchLib_RegisterLargeButtonReleaseSink(PFN_CAP_TOUCH_LIB_EVENT_SINK_VOID_VOID pfnEventSink)
{
if(0 == pfnEventSink)
{
mCapTouchLib_tLargeButton.pfnReleaseEventSink = CapTouchLib_DefaultEventSink;
}
else
{
mCapTouchLib_tLargeButton.pfnReleaseEventSink = pfnEventSink;
}
}
void CapTouchLib_RegisterSmallButtonPressSink(PFN_CAP_TOUCH_LIB_EVENT_SINK_VOID_VOID pfnEventSink)
{
if(0 == pfnEventSink)
{
mCapTouchLib_tSmallButton.pfnPressEventSink = CapTouchLib_DefaultEventSink;
}
else
{
mCapTouchLib_tSmallButton.pfnPressEventSink = pfnEventSink;
}
}
void CapTouchLib_RegisterSmallButtonReleaseSink(PFN_CAP_TOUCH_LIB_EVENT_SINK_VOID_VOID pfnEventSink)
{
if(0 == pfnEventSink)
{
mCapTouchLib_tSmallButton.pfnReleaseEventSink = CapTouchLib_DefaultEventSink;
}
else
{
mCapTouchLib_tSmallButton.pfnReleaseEventSink = pfnEventSink;
}
}
uint8_t CapTouchLib_EnableSmallButton(void)
{
if((mCapTouchLib_ptCurrentButton == &mCapTouchLib_tLargeButton) && (mCapTouchLib_tLargeButton.pfnCurrentState == CapTouchLib_State_InitButton))
{//large button is currently initing so we can not switch to the new button
return 1;
}
mCapTouchLib_tLargeButton.tNextButton = &mCapTouchLib_tSmallButton;
CapTouchLib_ReadButtonValue();//close out the current read
mCapTouchLib_ptCurrentButton = &mCapTouchLib_tSmallButton;
CapTouchLib_EnterInitButton();
return 0;
}
void CapTouchLib_DisableSmallButton(void)
{
mCapTouchLib_tLargeButton.tNextButton = &mCapTouchLib_tLargeButton;
}
//do nothing when not registered
void CapTouchLib_DefaultEventSink(void)
{
}
void CapTouchLib_EnterInitButton(void)
{
mCapTouchLib_ptCurrentButton->pfnCurrentState = CapTouchLib_State_InitButton;
}
void CapTouchLib_State_InitButton(void)
{
mCapTouchLib_ptCurrentButton->uiBaseSenseLevel = 0;
//go to next state on this button
CapTouchLib_EnterWaitForPress();
}
void CapTouchLib_EnterWaitForPress(void)
{
mCapTouchLib_ptCurrentButton->pfnCurrentState = CapTouchLib_State_WaitForPress;
mCapTouchLib_ptCurrentButton = mCapTouchLib_ptCurrentButton->tNextButton;
CapTouchLib_SetButtonForRead();
}
void CapTouchLib_State_WaitForPress(void)
{
//get a reading on the current button
CapTouchLib_ReadButtonValue();
if(mCapTouchLib_ptCurrentButton->uiBaseSenseLevel == 0)
{
mCapTouchLib_ptCurrentButton->uiBaseSenseLevel = mCapTouchLib_uiMeasurementCount;
}
else
{
if(mCapTouchLib_ptCurrentButton->uiBaseSenseLevel < mCapTouchLib_uiMeasurementCount)
// Handle baseline measurment for a base C decrease
{ // beyond baseline, i.e. cap decreased
mCapTouchLib_uiDeltaCount = 0; // Zero out delta for position determination
if(mCapTouchLib_ptCurrentButton->uiBaseSenseLevel+CAPTOUCHLIB_KEY_COMPARE < mCapTouchLib_uiMeasurementCount)
{
mCapTouchLib_uiMeasurementCount = mCapTouchLib_ptCurrentButton->uiBaseSenseLevel+CAPTOUCHLIB_KEY_COMPARE;
}
mCapTouchLib_ptCurrentButton->uiBaseSenseLevel = (mCapTouchLib_ptCurrentButton->uiBaseSenseLevel >> 1) + (mCapTouchLib_uiMeasurementCount >> 1); // Re-average baseline up quickly
}
else
{
mCapTouchLib_uiDeltaCount = mCapTouchLib_ptCurrentButton->uiBaseSenseLevel - mCapTouchLib_uiMeasurementCount; // Calculate delta: c_change
}
if (mCapTouchLib_uiDeltaCount > CAPTOUCHLIB_KEY_COMPARE) // Determine if each key is pressed per a preset threshold
{
//key pressed move to second check
mCapTouchLib_ptCurrentButton->pfnCurrentState = CapTouchLib_State_PressDelay;
}
else
{
mCapTouchLib_ptCurrentButton->uiBaseSenseLevel -= 1; // Adjust baseline down, should be slow to
}
}
mCapTouchLib_ptCurrentButton = mCapTouchLib_ptCurrentButton->tNextButton;
CapTouchLib_SetButtonForRead();
}
void CapTouchLib_State_PressDelay(void)
{
//get a reading on the current button
CapTouchLib_ReadButtonValue();
if(mCapTouchLib_ptCurrentButton->uiBaseSenseLevel < mCapTouchLib_uiMeasurementCount)
// Handle baseline measurment for a base C decrease
{ // beyond baseline, i.e. cap decreased
mCapTouchLib_uiDeltaCount = 0; // Zero out delta for position determination
if(mCapTouchLib_ptCurrentButton->uiBaseSenseLevel+CAPTOUCHLIB_KEY_COMPARE < mCapTouchLib_uiMeasurementCount)
{
mCapTouchLib_uiMeasurementCount = mCapTouchLib_ptCurrentButton->uiBaseSenseLevel+CAPTOUCHLIB_KEY_COMPARE;
}
mCapTouchLib_ptCurrentButton->uiBaseSenseLevel = (mCapTouchLib_ptCurrentButton->uiBaseSenseLevel >> 1) + (mCapTouchLib_uiMeasurementCount >> 1); // Re-average baseline up quickly
}
else
{
mCapTouchLib_uiDeltaCount = mCapTouchLib_ptCurrentButton->uiBaseSenseLevel - mCapTouchLib_uiMeasurementCount; // Calculate delta: c_change
}
if (mCapTouchLib_uiDeltaCount > CAPTOUCHLIB_KEY_COMPARE) // Determine if each key is pressed per a preset threshold
{
//key pressed call event sink
mCapTouchLib_ptCurrentButton->pfnPressEventSink();
CapTouchLib_EnterWaitForRelease();
}
else
{
//invalid press set back
mCapTouchLib_ptCurrentButton->pfnCurrentState = CapTouchLib_State_WaitForPress;
}
mCapTouchLib_ptCurrentButton = mCapTouchLib_ptCurrentButton->tNextButton;
CapTouchLib_SetButtonForRead();
}
void CapTouchLib_EnterWaitForRelease(void)
{
mCapTouchLib_ptCurrentButton->pfnCurrentState = CapTouchLib_State_WaitForRelease;
mCapTouchLib_ptCurrentButton = mCapTouchLib_ptCurrentButton->tNextButton;
CapTouchLib_SetButtonForRead();
}
void CapTouchLib_State_WaitForRelease(void)
{
//get a reading on the current button
CapTouchLib_ReadButtonValue();
if(mCapTouchLib_ptCurrentButton->uiBaseSenseLevel > mCapTouchLib_uiMeasurementCount)
// Handle baseline measurment for a base C decrease
{ // beyond baseline, i.e. cap decreased
mCapTouchLib_uiDeltaCount = 0; // Zero out delta for position determination
if(mCapTouchLib_ptCurrentButton->uiBaseSenseLevel > CAPTOUCHLIB_KEY_COMPARE + mCapTouchLib_uiMeasurementCount)
{
mCapTouchLib_uiMeasurementCount = mCapTouchLib_ptCurrentButton->uiBaseSenseLevel-CAPTOUCHLIB_KEY_COMPARE;
}
mCapTouchLib_ptCurrentButton->uiBaseSenseLevel = (mCapTouchLib_ptCurrentButton->uiBaseSenseLevel >> 1) + (mCapTouchLib_uiMeasurementCount >> 1); // Re-average baseline up quickly
}
else
{
mCapTouchLib_uiDeltaCount = mCapTouchLib_ptCurrentButton->uiBaseSenseLevel - mCapTouchLib_uiMeasurementCount; // Calculate delta: c_change
}
if (mCapTouchLib_uiDeltaCount > CAPTOUCHLIB_KEY_COMPARE) // Determine if each key is pressed per a preset threshold
{
mCapTouchLib_ptCurrentButton->pfnCurrentState = CapTouchLib_State_ReleaseDelay;
}
else
{
mCapTouchLib_ptCurrentButton->uiBaseSenseLevel -= 1; // Adjust baseline down, should be slow to
}
mCapTouchLib_ptCurrentButton = mCapTouchLib_ptCurrentButton->tNextButton;
CapTouchLib_SetButtonForRead();
}
void CapTouchLib_State_ReleaseDelay(void)
{
//get a reading on the current button
CapTouchLib_ReadButtonValue();
if(mCapTouchLib_ptCurrentButton->uiBaseSenseLevel > mCapTouchLib_uiMeasurementCount)
// Handle baseline measurment for a base C decrease
{ // beyond baseline, i.e. cap decreased
mCapTouchLib_uiDeltaCount = 0; // Zero out delta for position determination
if(mCapTouchLib_ptCurrentButton->uiBaseSenseLevel > CAPTOUCHLIB_KEY_COMPARE + mCapTouchLib_uiMeasurementCount)
{
mCapTouchLib_uiMeasurementCount = mCapTouchLib_ptCurrentButton->uiBaseSenseLevel-CAPTOUCHLIB_KEY_COMPARE;
}
mCapTouchLib_ptCurrentButton->uiBaseSenseLevel = (mCapTouchLib_ptCurrentButton->uiBaseSenseLevel >> 1) + (mCapTouchLib_uiMeasurementCount >> 1); // Re-average baseline up quickly
}
else
{
mCapTouchLib_uiDeltaCount = mCapTouchLib_ptCurrentButton->uiBaseSenseLevel - mCapTouchLib_uiMeasurementCount; // Calculate delta: c_change
}
if (mCapTouchLib_uiDeltaCount > CAPTOUCHLIB_KEY_COMPARE) // Determine if each key is pressed per a preset threshold
{
//key released call event sink
mCapTouchLib_ptCurrentButton->pfnReleaseEventSink();
CapTouchLib_EnterWaitForPress();
}
else
{
//invalid release set back
mCapTouchLib_ptCurrentButton->pfnCurrentState = CapTouchLib_State_WaitForRelease;
mCapTouchLib_ptCurrentButton->uiBaseSenseLevel -= 1; // Adjust baseline down, should be slow to
}
mCapTouchLib_ptCurrentButton = mCapTouchLib_ptCurrentButton->tNextButton;
CapTouchLib_SetButtonForRead();
}
void CapTouchLib_State_Disabled(void)
{
//do nothing
}
void CapTouchLib_SetButtonForRead(void)
{
// Context Save
mCapTouchLib_ubContextSaveSel = *(mCapTouchLib_ptCurrentButton->uiPortSelAddress);
mCapTouchLib_ubContextSaveSel2 = *(mCapTouchLib_ptCurrentButton->uiPortSel2Address);
// Configure Ports for relaxation oscillator
*(mCapTouchLib_ptCurrentButton->uiPortSelAddress) &= ~(mCapTouchLib_ptCurrentButton->uiPin);
*(mCapTouchLib_ptCurrentButton->uiPortSel2Address) |= (mCapTouchLib_ptCurrentButton->uiPin);
TA0CTL |= TACLR; // Clear Timer_A TAR
}
void CapTouchLib_ReadButtonValue(void)
{
//get a reading on the current button
TA0CCTL1 ^= CCIS0; // Create SW capture of CCR1
mCapTouchLib_uiMeasurementCount = TA0CCR1;
// Context Restore
*(mCapTouchLib_ptCurrentButton->uiPortSelAddress) = mCapTouchLib_ubContextSaveSel;
*(mCapTouchLib_ptCurrentButton->uiPortSel2Address) = mCapTouchLib_ubContextSaveSel2;
}
void CapTouchLib_Disable(void)
{
//set states to default
mCapTouchLib_tLargeButton.pfnCurrentState = CapTouchLib_State_Disabled;
mCapTouchLib_tSmallButton.pfnCurrentState = CapTouchLib_State_Disabled;
}
void CapTouchLib_Enable(void)
{
//set states to init
mCapTouchLib_tLargeButton.pfnCurrentState = CapTouchLib_State_InitButton;
mCapTouchLib_tSmallButton.pfnCurrentState = CapTouchLib_State_InitButton;
}
| 744a0a895a73f41c6149826c3b31d9fea7397de1 | [
"Markdown",
"C",
"Makefile",
"INI"
] | 16 | INI | AndrewTaft/MSP430_FireFlyJar | 955d312cf75df1631d71b0eaa0c54f885005bf69 | 948ab8868d06a96645f35aaf4f4e02bd1f598308 |
refs/heads/master | <repo_name>sadh/BUTP<file_sep>/README.md
BUTP is a TCP like UDP based socket implemented with flow cortrol, error control and congestion control<br />
====
<br /><br />Usage:<br />
//For server<br />
BUTPSocket socket = new BUTPSocket(9001);<br />
socket.accept();<br />
socket.receive(bytearray);<br />
socket.close();<br /><br />
//For client<br />
BUTPSocket socket = new BUTPSocket('server IP',server port):<br />
socket.connect();<br />
socket.send(bytearray);<br />
socket.close();<br /><file_sep>/src/edu/saib10/BUTPSocket.java
/**
* This class is the implementation of BUTP socket.
*
*/
package edu.saib10;
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
import java.net.SocketException;
import java.net.UnknownHostException;
import java.nio.ByteBuffer;
import java.util.Date;
import java.util.Random;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* BUTP socket class
* @author <NAME>
* @version 1.0
* @since 2012-03-05
*/
public class BUTPSocket {
private DatagramSocket socket;
private DatagramPacket send_packet, recv_packet;
private byte[] recv, send, BUTPSegment;
private int cwnd = 0, rwnd = 0, sequence, acknowledge,seq_ack,seq_tr,seq_rec;
private short checksum;
private BUTPPacket send_pkt, recv_pkt;
private short src_port, dst_port;
private InetAddress dst_addr;
private double RTTm, RTTs, RTTd;
private long RTO = 3000;
private static final long RTO_TIMEOUT = 60000, CONNECTIONTIMEOUT = 180000;
private static final int MAXWINDOWSIZE = 65535, INITIALWINDOW = 2840;
private byte flags, retransmission = 0;
private long send_time, recv_time;
private static final byte SYN_PSH = 0x00;
private static final byte SYN = 0x01;
private static final byte ACK = 0x02;
private static final byte SYN_ACK = 0x03;
private static final byte RST = 0x04;
private static final byte PSH = 0x08;
private static final byte FIN = 0x10;
private static final byte PSH_FIN = 0x18;
private static final int SEGMENT_SIZE = 1420;
private static boolean slowstart, congestion;
private static final Logger logger = Logger.getLogger(BUTPSocket.class.getName());
private static ExecutorService service;
/**
* Initiate client socket
* @param address Internet address of the receiver
* @param port Listening port address of the receiver
* @throws SocketException
* @throws UnknownHostException
* @throws IOException
*/
public BUTPSocket(String address, short port) throws SocketException, UnknownHostException, IOException {
dst_port = port;
Random rnd = new Random();
src_port = (short) (rnd.nextInt(9999) + 1);
dst_addr = InetAddress.getByName(address);
socket = new DatagramSocket(src_port);
service = Executors.newSingleThreadExecutor();
sequence = 0;
acknowledge = 0;
flags = SYN;
logger.log(Level.INFO,
"Initiating BUTP client socket-- Src:{0}:Dst:{1}:{2}",
new Object[]{src_port, dst_addr, dst_port});
}
/**
* Initiate server socket
* @param port Listening port of the receiver
* @throws SocketException
* @throws IOException
*/
public BUTPSocket(short port) throws SocketException {
src_port = port;
socket = new DatagramSocket(src_port);
service = Executors.newSingleThreadExecutor();
sequence = 0;
acknowledge = 0;
logger.log(Level.INFO, "Initiating server socket:Src:{0}", new Object[]{src_port});
}
/**
* Connect BUTP socket
*/
public void connect() throws IOException {
send(flags);
logger.log(Level.INFO, "Sending connection request");
Future future = service.submit(new Runnable() {
@Override
public void run() {
try {
receive(false);
} catch (IOException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "Socket Error.", ex);
}
}
});
try {
future.get(CONNECTIONTIMEOUT, TimeUnit.MILLISECONDS);
} catch (InterruptedException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "Interrupted.", ex);
} catch (ExecutionException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "Execution failed.", ex);
} catch (TimeoutException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "Connection timeout.");
future.cancel(true);
close();
System.exit(1);
}
flags = recv_pkt.getFlags();
if (flags == SYN_ACK) {
cwnd = recv_pkt.getWindowSize();
flags = SYN_PSH;
logger.log(Level.INFO,
"connection established with: {0}:{1}, window size: {2}",
new Object[]{dst_addr, dst_port, MAXWINDOWSIZE});
send(flags);
}
}
/**
* Accept incoming BUTP connection
*/
public void accept() throws IOException {
logger.info("waiting for connection.");
receive(false);
flags = recv_pkt.getFlags();
logger.log(Level.INFO, "Connection request recieved : flags:{0}", flags);
if (flags == SYN) {
rwnd = recv_pkt.getWindowSize();
cwnd = Math.min(cwnd, rwnd);
dst_addr = recv_packet.getAddress();
dst_port = (short) recv_packet.getPort();
logger.log(Level.INFO, "Request recieved from : {0}:{1}", new Object[]{dst_addr, dst_port});
flags = SYN_ACK;
send(flags);
Future future = service.submit(new Runnable() {
@Override
public void run() {
try {
receive(false);
} catch (IOException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "Receive failed.", ex);
}
}
});
try {
future.get(RTO, TimeUnit.MILLISECONDS);
} catch (InterruptedException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "Interrupted", ex);
} catch (ExecutionException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "Execution failed.", ex);
} catch (TimeoutException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "Connection timeout");
future.cancel(true);
close();
System.exit(1);
}
RTTm = (recv_time - send_time) * 2;
RTTs = RTTm;
RTTd = RTTm / 2;
flags = recv_pkt.getFlags();
if (flags == SYN_PSH) {
logger.log(Level.INFO, "Connection ready for transmition , RTT: {0}ms", RTTs);
}
}
}
/**
* Send flag packet SYN or FIN etc.
* @param flags for sending flag bytes
* @throws IOException
*/
private void send(byte flags) throws IOException {
this.flags = flags;
send_pkt = new BUTPPacket(cwnd, flags);
send = send_pkt.getBUTPByteArray();
send_packet = new DatagramPacket(send, send.length, dst_addr, dst_port);
send_time = System.currentTimeMillis();
logger.log(Level.INFO, "Sending flags at: {0}, flags: {1}", new Object[]{new Date(send_time), flags});
socket.send(send_packet);
}
/**
* Send acknowledgment packet
* @param acknowledge requesting next segment
* @param flags acknowledgment flag byte
* @throws IOException
*/
private void send(int acknowledge, byte flags) throws IOException {
this.acknowledge = acknowledge;
send_pkt = new BUTPPacket(acknowledge, cwnd, flags);
send = send_pkt.getBUTPByteArray();
send_packet = new DatagramPacket(send, send.length, dst_addr, dst_port);
send_time = System.currentTimeMillis();
logger.log(Level.INFO, "Sending acknowledge at {0}", new Date(send_time));
socket.send(send_packet);
}
/**
* Send BUTP segments.
* @param BUTP_segment BUTP segment.
* @param flag flag byte.
*/
private void send(byte[] BUTP_segment, byte flag) throws IOException {
send_pkt = new BUTPPacket(BUTP_segment, sequence, cwnd, flag);
send = send_pkt.getBUTPByteArray();
send_packet = new DatagramPacket(send, send.length, dst_addr, dst_port);
logger.log(Level.INFO, "Sending packet sequence: {0}", sequence);
socket.send(send_packet);
}
/**
* Send users data
* @param send_data Senders data byte array
* @throws IOException
*/
public final void send(byte[] send_data) throws IOException {
ByteBuffer buffer = ByteBuffer.wrap(send_data);
sequence = 0;
acknowledge = 0;
seq_ack = 0;
seq_tr = 0;
flags = PSH;
cwnd = INITIALWINDOW;
slowstart = true;
congestion = false;
byte[] BUTP_segment;
int sent = 0;
Future future = null;
while (buffer.hasRemaining()) {
if (sent <= cwnd) {
if (SEGMENT_SIZE > buffer.remaining()) {
BUTP_segment = new byte[buffer.remaining()];
sent = sent + buffer.remaining();
flags = PSH_FIN;
} else {
BUTP_segment = new byte[SEGMENT_SIZE];
sent = sent + SEGMENT_SIZE;
}
logger.log(Level.INFO, "Sent bytes: {0} bytes, window size: {1}", new Object[]{sent, cwnd});
sequence = buffer.position();
buffer.get(BUTP_segment);
send(BUTP_segment, flags);
seq_tr = sequence;
if(flags != PSH_FIN){
future = service.submit(new Runnable() {
@Override
public void run() {
try {
receive(false);
calculate_RTO();
acknowledge = recv_pkt.getAcknowledgeNumber();
if((acknowledge > seq_ack) && (acknowledge <= (seq_ack + cwnd))){
seq_ack = acknowledge;
}
logger.log(Level.INFO, "Acknowledgement received: {0}", new Object[]{acknowledge});
} catch (IOException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "Socket error: ", ex);
}
}
});
if(seq_ack == acknowledge){
sent -= SEGMENT_SIZE;
buffer.position(seq_ack);
}
try {
future.get(RTO, TimeUnit.MILLISECONDS);
if ((retransmission == 0) && (cwnd < (MAXWINDOWSIZE / 2)) && slowstart) {
cwnd *= 2;
} else if ((retransmission == 0) && (cwnd < (MAXWINDOWSIZE - 14200)) && congestion) {
cwnd += 14200;
} else if (retransmission == 3) {
slowstart = true;
congestion = false;
cwnd = INITIALWINDOW;
}
retransmission = 0;
} catch (InterruptedException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, null, ex);
} catch (ExecutionException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, null, ex);
}catch (TimeoutException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "RTO expired");
future.cancel(true);
RTO = RTO * 2;
if (retransmission < 3) {
slowstart = false;
congestion = true;
retransmission++;
} else {
future.cancel(true);
close();
System.exit(1);
}
buffer.position(seq_ack);
sent -= SEGMENT_SIZE;
}
}
}
}
}
/**
* Receiving single packet
* @param rto RTO enabled
* @throws IOException
*/
private void receive(boolean payload) throws IOException {
if (payload) {
recv = new byte[BUTPPacket.PAYLOAD + BUTPPacket.BUTP_HEADER];
} else {
recv = new byte[BUTPPacket.BUTP_HEADER];
}
recv_packet = new DatagramPacket(recv, recv.length);
socket.receive(recv_packet);
recv_time = System.currentTimeMillis();
recv = recv_packet.getData();
recv_pkt = new BUTPPacket();
recv_pkt.setBUTPByteArray(recv);
}
/**
* Receive users data
* @param receive_data the data byte array
* @throws IOException
*/
public final void receive(byte[] receive_data) throws IOException {
ByteBuffer buffer = ByteBuffer.wrap(receive_data);
buffer.clear();
sequence = 0;
acknowledge = 0;
rwnd = INITIALWINDOW;
logger.log(Level.INFO, "Start receiving data..");
int received = 0;
while ((flags == PSH) || (flags != PSH_FIN)) {
Future future = service.submit(new Runnable() {
@Override
public void run() {
try {
receive(true);
} catch (IOException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "Socket Error.", ex);
}
}
});
try {
future.get(CONNECTIONTIMEOUT, TimeUnit.MILLISECONDS);
checksum = recv_pkt.computeCheckSum();
logger.log(Level.INFO, "Computed checksum:{0} : Header checksum: {1} ", new Object[]{checksum, recv_pkt.getCheckSum()});
if (checksum == recv_pkt.getCheckSum()) {
sequence = recv_pkt.getSequenceNumber();
flags = recv_pkt.getFlags();
logger.log(Level.INFO,
"Receiving seqence: {0}, Acknowledged:{1}, Flags: {2}",
new Object[]{sequence, acknowledge, flags});
if (sequence == acknowledge) {
BUTPSegment = recv_pkt.getBUTPPayload();
received = received + BUTPSegment.length;
buffer.position(sequence);
int length = BUTPSegment.length;
if (flags == PSH_FIN) {
length = buffer.remaining();
}
buffer.put(BUTPSegment, 0, length);
acknowledge = buffer.position();
logger.log(Level.INFO,
"Received bytes: {0} bytes, window size:{1}",
new Object[]{received, rwnd});
if (flags != PSH_FIN){
logger.log(Level.INFO, "Sending acknowledge:- {0}", acknowledge);
send(acknowledge, ACK);
}
} else {
logger.log(Level.INFO,
"Discarded : received: {0}, Acknowledged:{1}, Flags: {2}",
new Object[]{sequence, acknowledge, flags});
send(acknowledge, ACK);
}
} else {
logger.log(Level.INFO, "Packet corrupted.");
send(acknowledge, ACK);
}
} catch (InterruptedException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "Interrupted.", ex);
} catch (ExecutionException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "receive failed.", ex);
} catch (TimeoutException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "Connection timeout.");
future.cancel(true);
close();
System.exit(1);
}
}
}
/**
* Implementation of Karn's Algorithm for RTO calculation
*/
private void calculate_RTO() {
RTTm = (recv_time - send_time) * 2;
RTTs = (0.875 * RTTs) + (0.125 * RTTm);
RTTd = ((0.75 * RTTd) + (0.25 * (double) Math.abs(RTTs - RTTm)));
RTO = (long) (RTTs + 4 * RTTd);
if (RTO < 1000) {
RTO = 1000;
}if(RTO > RTO_TIMEOUT){
try {
close();
return;
} catch (IOException ex) {
Logger.getLogger(BUTPSocket.class.getName()).log(Level.SEVERE, "RTO too long.", ex);
}
}
logger.log(Level.INFO, "Current RTT: {0}ms, Retransmition timeout: {1}ms, RTT variance: {2}ms", new Object[]{RTTs, RTO, RTTd});
}
/**
* Close the BUTP connection
* @throws IOException
*/
public void close() throws IOException {
logger.log(Level.INFO, "Closing connection.");
service.shutdownNow();
socket.close();
}
}
| d0e7541a5a55da2c1a0d143a3dda0c77fb8fbcd5 | [
"Markdown",
"Java"
] | 2 | Markdown | sadh/BUTP | 5636763f00cbc86fba4ade3c7b21b59aca39f108 | 5d45ec55918b7eaa20e44b403e5e2f6d34f593a8 |
refs/heads/master | <file_sep>use ncollide::query::{RayCast, RayIntersection, Ray};
use nalgebra::{Point3, Vector3, Isometry3};
use flight::vr::{Trackable, ViveController};
use std::collections::BinaryHeap;
use std::sync::{Arc, Mutex};
use std::cmp::{Ord, PartialOrd, PartialEq, Ordering};
pub struct VrGuru {
pub primary: ControllerGuru,
pub secondary: ControllerGuru,
}
impl VrGuru {
pub fn new(primary: &ViveController, secondary: &ViveController) -> VrGuru {
VrGuru {
primary: ControllerGuru {
data: ViveController {
.. *primary
},
queries: BinaryHeap::new(),
blocked: false,
laser_toi: None,
},
secondary: ControllerGuru {
data: ViveController {
.. *secondary
},
queries: BinaryHeap::new(),
blocked: false,
laser_toi: None,
},
}
}
pub fn apply(self) {
self.primary.apply();
self.secondary.apply();
}
}
struct ControllerQuery {
hit: RayIntersection<Vector3<f32>>,
reply: PointingReply,
stop: bool,
}
impl PartialEq for ControllerQuery {
fn eq(&self, other: &ControllerQuery) -> bool {
Arc::ptr_eq(&self.reply.0, &other.reply.0)
}
}
impl Eq for ControllerQuery {}
impl Ord for ControllerQuery {
fn cmp(&self, other: &ControllerQuery) -> Ordering {
self.partial_cmp(other).expect("TOI can't be NaN")
}
}
impl PartialOrd for ControllerQuery {
fn partial_cmp(&self, other: &ControllerQuery) -> Option<Ordering> {
other.hit.toi.partial_cmp(&self.hit.toi)
}
}
pub struct ControllerGuru {
pub data: ViveController,
pub laser_toi: Option<f32>,
queries: BinaryHeap<ControllerQuery>,
blocked: bool,
}
pub type PointingReply = Anywhere<Option<RayIntersection<Vector3<f32>>>>;
impl ControllerGuru {
pub fn laser<S: RayCast<Point3<f32>, Isometry3<f32>>>(
&mut self,
pos: &Isometry3<f32>,
shape: &S,
) {
let ray = Ray::new(self.data.origin(), self.data.pointing());
self.laser_toi = match (shape.toi_with_ray(pos, &ray, true), self.laser_toi) {
(Some(t), Some(o)) if t < o => Some(t),
(Some(t), None) => Some(t),
(_, o) => o,
};
}
pub fn pointing<S: RayCast<Point3<f32>, Isometry3<f32>>>(
&mut self,
pos: &Isometry3<f32>,
shape: &S,
stops: bool,
)
-> PointingReply
{
if !self.blocked {
let ray = Ray::new(self.data.origin(), self.data.pointing());
if let Some(i) = shape.toi_and_normal_with_ray(pos, &ray, true) {
let reply = Anywhere::new();
self.queries.push(ControllerQuery {
hit: i,
reply: reply.clone(),
stop: stops,
});
return reply;
}
}
Anywhere::with(None)
}
pub fn block_pointing(&mut self) {
self.blocked = true;
for q in self.queries.drain() {
q.reply.put(None);
}
}
pub fn apply(mut self) {
while let Some(q) = self.queries.pop() {
q.reply.put(Some(q.hit));
if q.stop { break; }
}
for q in self.queries {
q.reply.put(None);
}
}
}
pub struct Anywhere<T>(Arc<Mutex<Option<T>>>);
impl<T> Clone for Anywhere<T> {
fn clone(&self) -> Anywhere<T> {
Anywhere(self.0.clone())
}
}
impl<T> Anywhere<T> {
pub fn new() -> Anywhere<T> {
From::from(None)
}
pub fn with(v: T) -> Anywhere<T> {
From::from(Some(v))
}
pub fn take(&self) -> Option<T> {
self.0.lock().unwrap().take()
}
pub fn put(&self, v: T) {
*self.0.lock().unwrap() = Some(v);
}
pub fn expect(&self, msg: &str) -> T {
self.take().expect(msg)
}
}
impl<T> From<Option<T>> for Anywhere<T> {
fn from(v: Option<T>) -> Anywhere<T> {
Anywhere(Arc::new(Mutex::new(v)))
}
}
<file_sep>use gfx::{self, Factory};
use gfx::traits::FactoryExt;
use nalgebra::{self as na, Point3, Point2, Vector3, Similarity3, Isometry3, Translation3, UnitQuaternion};
use ncollide::shape::{Cuboid3, Plane};
use flight::{Texture, Light, PbrMesh, Error};
use flight::mesh::*;
use flight::load;
use flight::draw::{DrawParams, Painter, SolidStyle, PbrStyle, PbrMaterial};
use flight::vr::{primary, secondary, VrMoment, ViveController, Trackable};
use interact::{VrGuru};
pub const NEAR_PLANE: f64 = 0.1;
pub const FAR_PLANE: f64 = 75.;
pub const BACKGROUND: [f32; 4] = [0.529, 0.808, 0.980, 1.0];
const PI: f32 = ::std::f32::consts::PI;
const PI2: f32 = 2. * PI;
pub struct AppMats<R: gfx::Resources> {
plastic: PbrMaterial<R>,
floor: PbrMaterial<R>,
dark_plastic: PbrMaterial<R>,
blue_plastic: PbrMaterial<R>,
}
impl<R: gfx::Resources> AppMats<R> {
pub fn new<F: Factory<R> + FactoryExt<R>>(f: &mut F) -> Result<Self, Error> {
use gfx::format::*;
Ok(AppMats {
plastic: PbrMaterial {
normal: Texture::<_, (R8_G8_B8_A8, Unorm)>::uniform_value(f, [0x80, 0x80, 0xFF, 0xFF])?,
albedo: Texture::<_, (R8_G8_B8_A8, Srgb)>::uniform_value(f, [0x60, 0x60, 0x60, 0xFF])?,
metalness: Texture::<_, (R8, Unorm)>::uniform_value(f, 0x00)?,
roughness: Texture::<_, (R8, Unorm)>::uniform_value(f, 0x20)?,
},
floor: PbrMaterial {
normal: Texture::<_, (R8_G8_B8_A8, Unorm)>::uniform_value(f, [0x80, 0x80, 0xFF, 0xFF])?,
albedo: Texture::<_, (R8_G8_B8_A8, Srgb)>::uniform_value(f, [0xA0, 0xA0, 0xA0, 0xFF])?,
metalness: Texture::<_, (R8, Unorm)>::uniform_value(f, 0xFF)?,
roughness: Texture::<_, (R8, Unorm)>::uniform_value(f, 0x40)?,
},
dark_plastic: PbrMaterial {
normal: Texture::<_, (R8_G8_B8_A8, Unorm)>::uniform_value(f, [0x80, 0x80, 0xFF, 0xFF])?,
albedo: Texture::<_, (R8_G8_B8_A8, Srgb)>::uniform_value(f, [0x20, 0x20, 0x20, 0xFF])?,
metalness: Texture::<_, (R8, Unorm)>::uniform_value(f, 0x00)?,
roughness: Texture::<_, (R8, Unorm)>::uniform_value(f, 0x40)?,
},
blue_plastic: PbrMaterial {
normal: Texture::<_, (R8_G8_B8_A8, Unorm)>::uniform_value(f, [0x80, 0x80, 0xFF, 0xFF])?,
albedo: Texture::<_, (R8_G8_B8_A8, Srgb)>::uniform_value(f, [0x20, 0x20, 0xA0, 0xFF])?,
metalness: Texture::<_, (R8, Unorm)>::uniform_value(f, 0x00)?,
roughness: Texture::<_, (R8, Unorm)>::uniform_value(f, 0x40)?,
},
})
}
}
pub struct Model {
cubes: Vec<CubeModel>,
}
pub struct CubeModel {
grabbed: Option<Isometry3<f32>>,
pos: Isometry3<f32>,
radius: f32,
}
pub struct App<R: gfx::Resources> {
solid: Painter<R, SolidStyle<R>>,
pbr: Painter<R, PbrStyle<R>>,
controller: PbrMesh<R>,
line: Mesh<R, VertC, ()>,
floor: PbrMesh<R>,
cube: PbrMesh<R>,
mats: AppMats<R>,
primary: ViveController,
secondary: ViveController,
}
fn plane(rad: f32) -> MeshSource<VertN, ()> {
MeshSource {
verts: vec![
VertN { pos: [ rad, 0., rad], norm: [0., 1., 0.] },
VertN { pos: [-rad, 0., rad], norm: [0., 1., 0.] },
VertN { pos: [-rad, 0., -rad], norm: [0., 1., 0.] },
VertN { pos: [ rad, 0., rad], norm: [0., 1., 0.] },
VertN { pos: [-rad, 0., -rad], norm: [0., 1., 0.] },
VertN { pos: [ rad, 0., -rad], norm: [0., 1., 0.] },
],
inds: Indexing::All,
prim: Primitive::TriangleList,
mat: (),
}
}
fn bevel_cube(rad: f32, bev: f32) -> MeshSource<VertN, ()> {
let verts = vec![
VertN { pos: [rad, -(rad - bev), -(rad - bev)], norm: [1., 0., 0.] },
VertN { pos: [(rad - bev), -rad, -(rad - bev)], norm: [0., -1., 0.] },
VertN { pos: [(rad - bev), -(rad - bev), -rad], norm: [0., 0., -1.] },
VertN { pos: [rad, -(rad - bev), (rad - bev)], norm: [1., 0., 0.] },
VertN { pos: [(rad - bev), -(rad - bev), rad], norm: [0., 0., 1.] },
VertN { pos: [(rad - bev), -rad, (rad - bev)], norm: [0., -1., 0.] },
VertN { pos: [-(rad - bev), -(rad - bev), rad], norm: [0., 0., 1.] },
VertN { pos: [-rad, -(rad - bev), (rad - bev)], norm: [-1., 0., 0.] },
VertN { pos: [-(rad - bev), -rad, (rad - bev)], norm: [0., -1., 0.] },
VertN { pos: [-(rad - bev), -(rad - bev), -rad], norm: [0., 0., -1.] },
VertN { pos: [-(rad - bev), -rad, -(rad - bev)], norm: [0., -1., 0.] },
VertN { pos: [-rad, -(rad - bev), -(rad - bev)], norm: [-1., 0., 0.] },
VertN { pos: [(rad - bev), (rad - bev), -rad], norm: [0., 0., -1.] },
VertN { pos: [(rad - bev), rad, -(rad - bev)], norm: [0., 1., 0.] },
VertN { pos: [rad, (rad - bev), -(rad - bev)], norm: [1., 0., 0.] },
VertN { pos: [(rad - bev), (rad - bev), rad], norm: [0., 0., 1.] },
VertN { pos: [rad, (rad - bev), (rad - bev)], norm: [1., 0., 0.] },
VertN { pos: [(rad - bev), rad, (rad - bev)], norm: [0., 1., 0.] },
VertN { pos: [-rad, (rad - bev), (rad - bev)], norm: [-1., 0., 0.] },
VertN { pos: [-(rad - bev), (rad - bev), rad], norm: [0., 0., 1.] },
VertN { pos: [-(rad - bev), rad, (rad - bev)], norm: [0., 1., 0.] },
VertN { pos: [-rad, (rad - bev), -(rad - bev)], norm: [-1., 0., 0.] },
VertN { pos: [-(rad - bev), rad, -(rad - bev)], norm: [0., 1., 0.] },
VertN { pos: [-(rad - bev), (rad - bev), -rad], norm: [0., 0., -1.] },
];
let inds = vec![3-1, 24-1, 13-1, 6-1, 11-1, 2-1, 19-1, 12-1, 8-1, 23-1,
18-1, 14-1, 16-1, 7-1, 5-1, 1-1, 2-1, 3-1, 4-1, 5-1, 6-1, 7-1, 8-1, 9-1,
10-1, 11-1, 12-1, 13-1, 14-1, 15-1, 16-1, 17-1, 18-1, 19-1, 20-1, 21-1,
22-1, 23-1, 24-1, 4-1, 2-1, 1-1, 11-1, 3-1, 2-1, 13-1, 1-1, 3-1, 7-1, 6-1,
5-1, 17-1, 5-1, 4-1, 12-1, 9-1, 8-1, 20-1, 8-1, 7-1, 22-1, 10-1, 12-1, 18-1,
15-1, 14-1, 24-1, 14-1, 13-1, 21-1, 16-1, 18-1, 23-1, 19-1, 21-1, 15-1, 4-1,
1-1, 3-1, 10-1, 24-1, 6-1, 9-1, 11-1, 19-1, 22-1, 12-1, 23-1, 21-1, 18-1,
16-1, 20-1, 7-1, 4-1, 6-1, 2-1, 11-1, 10-1, 3-1, 13-1, 15-1, 1-1, 7-1, 9-1,
6-1, 17-1, 16-1, 5-1, 12-1, 11-1, 9-1, 20-1, 19-1, 8-1, 22-1, 24-1, 10-1,
18-1, 17-1, 15-1, 24-1, 23-1, 14-1, 21-1, 20-1, 16-1, 23-1, 22-1, 19-1,
15-1, 17-1, 4-1];
MeshSource {
verts: verts,
inds: Indexing::Inds(inds),
prim: Primitive::TriangleList,
mat: (),
}
}
impl<R: gfx::Resources> App<R> {
pub fn model(&self) -> Model {
Model {
cubes: (0i32..10).map(|i| {
let rad = 0.2 * (1. - i as f32 / 15.);
let theta = (i as f32) / 5. * PI;
CubeModel {
grabbed: None,
pos: Isometry3::from_parts(
Translation3::new(theta.sin() * 1., 0., theta.cos() * 1.),
UnitQuaternion::from_axis_angle(&Vector3::y_axis(), theta)
),
radius: rad,
}
}).collect(),
}
}
pub fn new<F: Factory<R> + FactoryExt<R>>(factory: &mut F) -> Result<Self, Error> {
// Setup Painters
let mut solid = Painter::new(factory)?;
solid.setup(factory, Primitive::LineList)?;
solid.setup(factory, Primitive::TriangleList)?;
let mut pbr: Painter<_, PbrStyle<_>> = Painter::new(factory)?;
pbr.setup(factory, Primitive::TriangleList)?;
let mat = AppMats::new(factory)?;
// Construct App
Ok(App {
solid: solid,
pbr: pbr,
controller: load::wavefront_file("assets/controller.obj")?
.compute_tan()
.with_material(mat.plastic.clone())
.upload(factory),
line: MeshSource {
verts: vec![
VertC { pos: [0., 0., 0.], color: [0.22, 0.74, 0.94] },
VertC { pos: [0., 0., -1.], color: [0.2, 0.28, 0.31] },
],
inds: Indexing::All,
prim: Primitive::LineList,
mat: (),
}.upload(factory),
cube: bevel_cube(1., 0.05)
.with_tex(Point2::new(0., 0.))
.compute_tan()
.with_material(mat.dark_plastic.clone())
.upload(factory),
floor: plane(5.)
.with_tex(Point2::new(0., 0.))
.compute_tan()
.with_material(mat.floor.clone())
.upload(factory),
mats: mat,
primary: ViveController {
is: primary(),
.. Default::default()
},
secondary: ViveController {
is: secondary(),
.. Default::default()
}
})
}
pub fn draw<C: gfx::CommandBuffer<R>>(
&mut self,
ctx: &mut DrawParams<R, C>,
vrm: &VrMoment,
model: &mut Model,
) {
match (self.primary.update(vrm), self.secondary.update(vrm)) {
(Ok(_), Ok(_)) => (),
_ => warn!("A not vive-like controller is connected"),
}
// Clear targets
ctx.encoder.clear_depth(&ctx.depth, FAR_PLANE as f32);
ctx.encoder.clear(&ctx.color, [BACKGROUND[0].powf(1. / 2.2), BACKGROUND[1].powf(1. / 2.2), BACKGROUND[2].powf(1. / 2.2), BACKGROUND[3]]);
// Config PBR lights
self.pbr.cfg(|s| {
s.ambient(BACKGROUND);
s.lights(&[
Light {
pos: vrm.stage * Point3::new((0. * PI2 / 3.).sin() * 2., 4., (0. * PI2 / 3.).cos() * 2.),
color: [1.0, 0.8, 0.8, 85.],
},
Light {
pos: vrm.stage * Point3::new((1. * PI2 / 3.).sin() * 2., 4., (1. * PI2 / 3.).cos() * 2.),
color: [0.8, 1.0, 0.8, 85.],
},
Light {
pos: vrm.stage * Point3::new((2. * PI2 / 3.).sin() * 2., 4., (2. * PI2 / 3.).cos() * 2.),
color: [0.8, 0.8, 1.0, 85.],
},
Light {
pos: vrm.stage * Point3::new(0., -8., 0.),
color: [1.0, 1.0, 1.0, 200.],
},
]);
});
// Draw & update cubes
let mut guru = VrGuru::new(&self.primary, &self.secondary);
let cube_partials: Vec<_> = model.cubes
.iter_mut()
.map(|model| {
if model.grabbed.is_some() && guru.primary.data.trigger > 0.5 {
guru.primary.block_pointing();
} else {
model.grabbed = None;
}
let cuboid = Cuboid3::new(Vector3::from_element(model.radius));
guru.primary.laser(&model.pos, &cuboid);
let reply = guru.primary.pointing(
&model.pos,
&cuboid,
true);
move |ctx: &mut DrawParams<R, C>, app: &mut App<R>| {
if let Some(_) = reply.expect("pointing not applied") {
// TODO: speed not delta
// Yank
if app.primary.pad_delta[1] < 0. && app.primary.pad[1] < 0. {
model.pos = Isometry3::from_parts(
Translation3::from_vector((app.primary.pose() *
Point3::new(0., 0., -0.1 -
model.radius)).coords), model.pos.rotation,
);
}
// TODO: speed not delta
// Push
if app.primary.pad_delta[1] > 0. && app.primary.pad[1] > 0. {
model.pos = Isometry3::from_parts(
Translation3::from_vector((app.primary.pose() *
Point3::new(0., 0., -2.5)).coords),
model.pos.rotation,
);
}
// Grab
if app.primary.trigger > 0.5
&& app.primary.trigger - app.primary.trigger_delta < 0.5 {
model.grabbed = Some(app.primary.pose().inverse() * model.pos);
}
}
// Update position
if let Some(off) = model.grabbed {
model.pos = app.primary.pose() * off;
app.pbr.draw(
ctx,
na::convert(Similarity3::from_isometry(model.pos, model.radius)),
&Mesh {
mat: app.mats.blue_plastic.clone(),
.. app.cube.clone()
},
);
} else {
app.pbr.draw(
ctx,
na::convert(Similarity3::from_isometry(model.pos, model.radius)),
&app.cube
);
}
}
})
.collect();
let stage = na::try_convert(vrm.stage).unwrap_or(na::one());
guru.primary.laser(&stage, &Plane::new(Vector3::y()));
let toi = guru.primary.laser_toi.unwrap_or(FAR_PLANE as f32).max(0.01);
guru.apply();
for mut p in cube_partials {
p(ctx, self);
}
// Draw controllers
for cont in vrm.controllers() {
self.pbr.draw(ctx, na::convert(cont.pose), &self.controller);
}
self.solid.draw(ctx, na::convert(
Similarity3::from_isometry(self.primary.pose(), toi)
), &self.line);
// Draw floor
self.pbr.draw(ctx, na::convert(stage), &self.floor);
}
}
<file_sep>[package]
name = "workbench"
version = "0.1.0"
authors = [
"<NAME> <<EMAIL>>",
]
[dependencies]
flight = { git="https://github.com/flight-rs/flight" }
clap = "*"
simplelog = "*"
glutin = "*"
gfx_window_glutin = "*"
gfx_device_gl = "*"
nalgebra = "*"
gfx = "*"
log = "*"
ncollide = "*"
<file_sep>#![allow(dead_code)]
use std::collections::VecDeque;
use std::sync::Arc;
/// Just an `f32`.
pub type Param = f32;
/// Just an `f32`.
pub type Time = f32;
/// Just an `f32`.
pub type DeltaTime = f32;
/// An animation state.
pub trait Animation<V>: Clone {
/// Get the current output of the animation.
fn now(&self) -> V;
/// Step the animation forward.
fn step(&mut self, dt: DeltaTime);
/// Ensure that the animation is in a normal form and will not behave in an
/// undefined way. If the animation is in a valid, nontrivial state, then
/// this should do nothing.
///
/// The most common invalid state is an ended (unchanging) `Animate`
/// variant which is not `Animate::Fixed`.
fn normalize(mut self) -> Self { self.step(0.); self }
/// Check if this animation has reached a steady state.
fn steady(&self) -> bool;
}
/// The main animation type. Provides a wide variety of animation functions.
#[derive(Clone)]
pub enum Animate<V: Mixable> {
/// Hold the given value.
Fixed(V),
/// Move from `a→b` over the next `t` seconds, then stop.
Slide(V, V, Time),
/// Move from `a→b`, outputting `a` after `s` seconds then `b` after `t` seconds.
Linear(V, V, Time, Time),
/// Move through the curve `a→b→c`, outputting `a` after `s` seconds then `c` after `t` seconds.
Quadratic(V, V, V, Time, Time),
/// Move through the curve `a→b→c→d`, outputting `a` after `s` seconds then `d` after `t` seconds.
Cubic(V, V, V, V, Time, Time),
/// Move from `a→b`, starting after `s` seconds then ending after `t` seconds.
BoundedLinear(V, V, Time, Time),
/// Move through the curve `a→b→c`, starting after `s` seconds then ending after `t` seconds.
BoundedQuadratic(V, V, V, Time, Time),
/// Move through the curve `a→b→c→d`, starting after `s` seconds then ending after `t` seconds.
BoundedCubic(V, V, V, V, Time, Time),
/// Switch from `a` to `b` after `t` seconds.
Switch(V, V, Time),
/// Switch from `a` to `b` between `s` seconds and `t` seconds from now.
SmoothSwitch(V, V, Time, Time),
/// Soft switch from `a` to `b` using parameter `e` between `s` seconds and `t` seconds from now.
SoftSwitch(V, V, i32, Time, Time),
/// Outputs `f(x)` starting with `x=t`.
Func(Arc<Fn(Time) -> V>, Time),
/// `f(x)` mixes between `a` and `b` starting with `x=t`.
MixFunc(Arc<Fn(Time) -> Time>, V, V, Time),
/// `f(v, dt)` is repeatedly applied to `v` every step.
StepFunc(Arc<Fn(V, DeltaTime) -> V>, V),
/// Play a sequence of animations.
Sequence(AnimateSequence<V>),
}
use self::Animate::*;
impl<V: Mixable> Animate<V> {
pub fn bounce_soft(a: V, b: V, duration: Time) -> Animate<V> {
MixFunc(Arc::new(move |t| {
let t = t / duration;
if t < 0. || t > 1. { return 0. }
let mut x = t * 2. - 1.;
x *= x;
x * x - 2. * x + 1. // x^4 - 2x^2 + 1
}), a, b, 0.)
}
pub fn bounce_hard(a: V, b: V, duration: Time) -> Animate<V> {
MixFunc(Arc::new(move |t| {
let t = t / duration;
if t < 0. || t > 1. { return 0. }
let x = t * 2. - 1.;
1. - (x * x) // -x^2 + 1
}), a, b, 0.)
}
#[inline]
fn do_step(self, dt: DeltaTime) -> Self {
match self {
Fixed(a) => Fixed(a),
Slide(a, b, t) => if dt > t {
Fixed(b)
} else {
Slide(V::linear(&a, &b, dt / t), b, t - dt)
},
Linear(a, b, s, t) => Linear(a, b, s - dt, t - dt),
Quadratic(a, b, c, s, t) => Quadratic(a, b, c, s - dt, t - dt),
Cubic(a, b, c, d, s, t) => Cubic(a, b, c, d, s - dt, t- dt),
BoundedLinear(a, b, s, t) => if dt > t {
Fixed(b)
} else {
BoundedLinear(a, b, s - dt, t - dt)
},
BoundedQuadratic(a, b, c, s, t) => if dt > t {
Fixed(c)
} else {
BoundedQuadratic(a, b, c, s - dt, t - dt)
},
BoundedCubic(a, b, c, d, s, t) => if dt > t {
Fixed(d)
} else {
BoundedCubic(a, b, c, d, s - dt, t - dt)
},
Switch(a, b, t) => if dt > t {
Fixed(b)
} else {
Switch(a, b, t - dt)
},
SmoothSwitch(a, b, s, t) => if dt > t {
Fixed(b)
} else {
SmoothSwitch(a, b, s - dt, t - dt)
},
SoftSwitch(a, b, e, s, t) => if dt > t {
Fixed(b)
} else {
SoftSwitch(a, b, e, s - dt, t - dt)
},
Func(f, s) => Func(f, s + dt),
MixFunc(f, a, b, s) => MixFunc(f, a, b, s + dt),
StepFunc(f, v) => { let v = f(v, dt); StepFunc(f, v) },
Sequence(mut seq) => { seq.step(dt); Sequence(seq) },
}
}
}
impl<V: Mixable> Animation<V> for Animate<V> {
fn now(&self) -> V {
match *self {
Fixed(ref x) => x.clone(),
Slide(ref x, _, _) => x.clone(),
Linear(ref a, ref b, s, t) => V::linear(a, b, s / (s - t)),
Quadratic(ref a, ref b, ref c, s, t) => V::quadratic(a, b, c, s / (s - t)),
Cubic(ref a, ref b, ref c, ref d, s, t) => V::cubic(a, b, c, d, s / (s - t)),
BoundedLinear(ref a, ref b, s, t) => if s > 0. {
a.clone()
} else {
V::linear(a, b, s / (s - t))
},
BoundedQuadratic(ref a, ref b, ref c, s, t) => if s > 0. {
a.clone()
} else {
V::quadratic(a, b, c, s / (s - t))
},
BoundedCubic(ref a, ref b, ref c, ref d, s, t) => if s > 0. {
a.clone()
} else {
V::cubic(a, b, c, d, s / (s - t))
},
Switch(ref a, _, _) => a.clone(),
SmoothSwitch(ref a, ref b, s, t) => if s > 0. {
a.clone()
} else {
let x = s / (s - t);
let xx = x * x;
V::linear(a, b, 3. * xx - 2. * xx * x)
},
SoftSwitch(ref a, ref b, e, s, t) => if s > 0. {
a.clone()
} else {
let x = s / (s - t);
let x = 1. - x;
V::linear(a, b, x.powi(e))
},
Func(ref f, t) => f(t),
MixFunc(ref f, ref a, ref b, t) => V::linear(a, b, f(t)),
StepFunc(_, ref v) => v.clone(),
Sequence(ref seq) => seq.now(),
}
}
fn step(&mut self, dt: DeltaTime) {
let ptr = self as *mut Self;
use std::ptr::*;
unsafe {
write(ptr, read(ptr).do_step(dt));
}
}
fn steady(&self) -> bool {
match self {
&Fixed(_) => true,
_ => false,
}
}
}
/// A sequence of different animations, each one lasting a given duration.
#[derive(Clone)]
pub struct AnimateSequence<V: Mixable> {
/// A queue of animations to play, and their durations.
pub queue: VecDeque<(Animate<V>, Time)>,
/// The value to hold once the queue is empty.
pub end: V,
}
impl<V: Mixable> AnimateSequence<V> {
pub fn new(end: V) -> AnimateSequence<V> {
AnimateSequence {
queue: VecDeque::new(),
end: end,
}
}
pub fn before(&mut self, time: Time, anim: Animate<V>) {
self.queue.push_front((anim, time));
}
}
impl<V: Mixable> Animation<V> for AnimateSequence<V> {
fn now(&self) -> V {
self.queue.get(0).map(|&(ref v, _)| v.now()).unwrap_or(self.end.clone())
}
fn step(&mut self, mut dt: DeltaTime) {
// Logic to step over multiple animations when the `dt` is large
let mut count = 0;
for &mut (ref mut a, ref mut t) in self.queue.iter_mut() {
if dt > *t {
// step into next animation, bringing residual `dt` forward
dt -= *t;
count += 1;
} else {
// step forward in current animation
a.step(dt);
*t -= dt;
break;
}
}
self.queue.drain(..count);
}
fn steady(&self) -> bool {
match self.queue.back() {
Some(a) => a.0.steady(),
None => true,
}
}
}
/// A type that can be animated.
pub trait Mixable: Sized + Clone {
/// Most animations are performed by mixing together various provided values,
/// so a mixer is needed.
type Mixer: Mixer<Self>;
/// Mix together 2 values with linear interpolation.
fn linear(a: &Self, b: &Self, t: Param) -> Self {
let mut acc = Self::Mixer::new();
acc.add(a, 1. - t);
acc.add(b, t);
acc.close()
}
/// Mix together 3 values with a quadratic bezier function.
fn quadratic(a: &Self, b: &Self, c: &Self, t: Param) -> Self {
let s = 1. - t;
let mut acc = Self::Mixer::new();
acc.add(a, s * s);
acc.add(b, 2. * s * t);
acc.add(c, t * t);
acc.close()
}
/// Mix together 4 values with a cubic bezier function.
fn cubic(a: &Self, b: &Self, c: &Self, d: &Self, t: Param) -> Self {
let s = 1. - t;
let mut acc = Self::Mixer::new();
acc.add(a, s * s * s);
acc.add(b, 3. * s * s * t);
acc.add(c, 3. * s * t * t);
acc.add(d, t * t * t);
acc.close()
}
/// Mix together an iterator of values and their weights. If there are no
/// values, or the weights do not sum to `1`, then the result is not well
/// defined.
fn mix<I: Iterator<Item=(Self, Param)>>(iter: I) -> Self {
let mut acc = Self::Mixer::new();
for (ref v, w) in iter {
acc.add(v, w);
}
acc.close()
}
}
/// Used to mix together several values with varying weights. The mixer is
/// not responsible for normalizing the given weights, if they do not sum to
/// `1` then the behavior of the mixer is not well defined.
pub trait Mixer<V>: Sized {
/// Instantiate a new mixer.
fn new() -> Self;
/// Mix a new value into the total.
fn add(&mut self, v: &V, weight: Param);
/// Finish mixing the values together. If no values have been added then
/// the result is not well defined.
fn close(self) -> V;
}
use nalgebra as na;
use nalgebra::*;
impl Mixer<Self> for f32 {
fn new() -> Self { 0. }
fn add(&mut self, v: &Self, weight: Param) { *self += v * weight }
fn close(self) -> Self { self }
}
impl Mixable for f32 { type Mixer = Self; }
impl Mixer<Self> for f64 {
fn new() -> Self { 0. }
fn add(&mut self, v: &Self, weight: Param) { *self += v * weight as f64 }
fn close(self) -> Self { self }
}
impl Mixable for f64 { type Mixer = Self; }
macro_rules! impl_mix {
(<$g:ident: $gb:path> $i:ty = $t:ty, || $n:expr, |$ep:ident| $e:expr, |$cp:ident| $c:expr) => {
impl<$g: $gb> Mixer<$i> for $t {
fn new() -> Self { $n }
fn add(&mut self, v: &$i, weight: Param) {
let $ep = v;
*self += $e * $g::from_f32(weight).unwrap();
}
fn close(self) -> $i { let $cp = self; $c }
}
impl<$g: $gb> Mixable for $i { type Mixer = $t; }
};
}
macro_rules! nalg_mix {
({$($a:path = $b:path),*$(,)*}, $g:ident, |$ep:ident| $e:expr, |$cp:ident| $c:expr) => (
$(impl_mix!(<$g: Real> $a = $b, || na::zero(), |$ep| $e, |$cp| $c);)*
)
}
nalg_mix!({
Vector1<F> = Vector1<F>,
Vector2<F> = Vector2<F>,
Vector3<F> = Vector3<F>,
Vector4<F> = Vector4<F>,
Vector5<F> = Vector5<F>,
Vector6<F> = Vector6<F>,
}, F, |v| v, |c| c);
nalg_mix!({
Matrix2<F> = Matrix2<F>,
Matrix3<F> = Matrix3<F>,
Matrix4<F> = Matrix4<F>,
Matrix5<F> = Matrix5<F>,
Matrix6<F> = Matrix6<F>,
}, F, |v| v, |c| c);
nalg_mix!({
Point1<F> = Vector1<F>,
Point2<F> = Vector2<F>,
Point3<F> = Vector3<F>,
Point4<F> = Vector4<F>,
Point5<F> = Vector5<F>,
Point6<F> = Vector6<F>,
}, F, |v| v.coords, |c| Point::from_coordinates(c));
nalg_mix!({
Translation2<F> = Vector2<F>,
Translation3<F> = Vector3<F>,
}, F, |v| v.vector, |c| Translation::from_vector(c));
impl_mix!(
<F: Real> Quaternion<F> = Quaternion<F>,
|| na::zero(),
|v| v,
|c| c);
impl_mix!(
<F: Real> UnitQuaternion<F> = Quaternion<F>,
|| na::zero(),
|v| v.unwrap(),
|c| Unit::try_new(c, F::default_epsilon()).unwrap_or(Unit::new_unchecked(c)));
type Isometry3Mixer<F> =
(<UnitQuaternion<F> as Mixable>::Mixer, <Translation3<F> as Mixable>::Mixer);
impl<F: Real> Mixer<Isometry3<F>> for Isometry3Mixer<F> {
fn new() -> Self {
(
<Quaternion<F> as Mixer<UnitQuaternion<F>>>::new(),
<Vector3<F> as Mixer<Translation3<F>>>::new())
}
fn add(&mut self, v: &Isometry3<F>, weight: Param) {
Mixer::add(&mut self.0, &v.rotation, weight);
Mixer::add(&mut self.1, &v.translation, weight);
}
fn close(self) -> Isometry3<F> {
Isometry3::from_parts(Mixer::close(self.1), Mixer::close(self.0))
}
}
impl<F: Real> Mixable for Isometry3<F> { type Mixer = Isometry3Mixer<F>; }
type Similarity3Mixer<F> =
(<Isometry3<F> as Mixable>::Mixer, <F as Mixable>::Mixer);
impl<F: Real + Mixable> Mixer<Similarity3<F>> for Similarity3Mixer<F> {
fn new() -> Self {
(Mixer::new(), Mixer::new())
}
fn add(&mut self, v: &Similarity3<F>, weight: Param) {
Mixer::add(&mut self.0, &v.isometry, weight);
Mixer::add(&mut self.1, &v.scaling(), weight);
}
fn close(self) -> Similarity3<F> {
Similarity3::from_isometry(Mixer::close(self.0), Mixer::close(self.1))
}
}
impl<F: Real + Mixable> Mixable for Similarity3<F> { type Mixer = Similarity3Mixer<F>; } | c466d1679c794cc60ac19b25acf2b1a494449a62 | [
"TOML",
"Rust"
] | 4 | Rust | CSM-Dream-Team/workbench | 02ac448cd057902f28baf11ec4c4d0d1ca513546 | 5d89bfc54673c11ffbccbf1d527022de46f71bb9 |
refs/heads/master | <repo_name>lx719679895/MiniProgramStudy<file_sep>/README.md
# MiniProgramStudy
微信小程序学习
## 小程序
### 什么是小程序?
1. 无需下载,用完即走(体积小,刚发布的小程序压缩包体积不能超过1M,2017年4月提升为2M)
### 小程序特点
1. 体积小
2. 同app进行互补,可以实现app的基本功能
3. 微信扫一扫或者搜索就可以去下载
4. 开发周期短,成本较低
### 适配方案
1. viewport适配 width=device-width
2. 单位: rpx
3. iphone6 1rpx=1物理像素=0.5px dpr=物理像素/设备独立像素=2
### 重要文件
1. wxml view结构--->html
2. wxss view样式--->css
3. js view行为--->js
4. json文件 数据&&配置
### 注册小程序 App ()
### 注册页面 Page ()
### 数据绑定
1. 在data中初始化页面需要的数据,在页面中可以直接使用
### 事件(冒泡事件 || 非冒泡事件)
1. 冒泡事件 bind+事件名
2. 非冒泡事件 catch+事件名
### 模板template
1. 定义:template 属性:name(标识模板)
2. 使用:template 属性:is(模板的name)
3. 引入模板结构:<import src="path" />
4. 引入模板样式:@import 'path'
5. 传参:data="{{...变量}}"
### 列表渲染
1. wx:for
2. wx:key 为每个个体元素进行标记,提高性能
3. 遍历的个体:item
4. 遍历的下标:index
### 本地缓存(setStorage,setStorageSync)
1. 缓存的是用户是否收藏当前文章,多篇文章缓存在一个key中,value是object对象{0:true,1:false}
2. 注意:
1. 缓存之前应该先去获取之前本地缓存的数据
2. 缓存的新数据是在原有数据的基础上修改的
3. 当页面加载的时候onLoad中获取本地缓存的数据
4. 如果storage中没有缓存过,通过key获取的value值为undefined!!
### 音乐播放
1. 如何知道音乐的播放或暂停
2. 将播放音乐的页面状态保存到appData中
### tabBar
1. app.json文件中配置
2. 跳转到tabBar使用wx.switchTab(Object object)
### 发送ajax请求实现
1. wx.request(Object object)<file_sep>/pages/movieDetail/movieDetail.js
// pages/movieDetail/movieDetail.js
let pageData = getApp()
Page({
/**
* 页面的初始数据
*/
data: {
detail: {},
castStr: ''
},
/**
* 生命周期函数--监听页面加载
*/
onLoad: function (options) {
let val = pageData.data.movieList[options.index]
let casts = val.casts
let castStr = ''
if (casts.length) {
casts.forEach(el => {
castStr += el.name + ' '
})
}
this.setData({
detail: val,
castStr
})
console.log(this.data.detail)
wx.setNavigationBarTitle({
title: val.title
})
},
/**
* 生命周期函数--监听页面初次渲染完成
*/
onReady: function () {
},
/**
* 生命周期函数--监听页面显示
*/
onShow: function () {
},
/**
* 生命周期函数--监听页面隐藏
*/
onHide: function () {
},
/**
* 生命周期函数--监听页面卸载
*/
onUnload: function () {
},
/**
* 页面相关事件处理函数--监听用户下拉动作
*/
onPullDownRefresh: function () {
},
/**
* 页面上拉触底事件的处理函数
*/
onReachBottom: function () {
},
/**
* 用户点击右上角分享
*/
onShareAppMessage: function () {
}
}) | 692e1ce39d3668add6d3d89c46ab3919bfd7eb4c | [
"Markdown",
"JavaScript"
] | 2 | Markdown | lx719679895/MiniProgramStudy | 78130a49019c547fe4db3b85c8c44bee19d21f35 | 3be822c486b26bbbb8d00bbe121ae4b99c10f3ce |