full_name
stringlengths 7
104
| description
stringlengths 4
725
⌀ | topics
stringlengths 3
468
⌀ | readme
stringlengths 13
565k
⌀ | label
int64 0
1
|
---|---|---|---|---|
mauron85/react-native-background-geolocation | Background and foreground geolocation plugin for React Native. Tracks user when app is running in background. | null | # @mauron85/react-native-background-geolocation
[](https://circleci.com/gh/mauron85/react-native-background-geolocation/tree/master)
[](https://issuehunt.io/r/mauron85/react-native-background-geolocation/)
## We're moving
Npm package is now [@mauron85/react-native-background-geolocation](https://www.npmjs.com/package/@mauron85/react-native-background-geolocation)!
## Submitting issues
All new issues should follow instructions in [ISSUE_TEMPLATE.md](https://raw.githubusercontent.com/mauron85/react-native-background-geolocation/master/ISSUE_TEMPLATE.md).
A properly filled issue report will significantly reduce number of follow up questions and decrease issue resolving time.
Most issues cannot be resolved without debug logs. Please try to isolate debug lines related to your issue.
Instructions for how to prepare debug logs can be found in section [Debugging](#debugging).
If you're reporting an app crash, debug logs might not contain all the necessary information about the cause of the crash.
In that case, also provide relevant parts of output of `adb logcat` command.
## Issue Hunt
Fund your issues or feature request to drag attraction of developers. Checkout our [issue hunt page](https://issuehunt.io/r/mauron85/react-native-background-geolocation/issues).
# Android background service issues
There are repeatedly reported issues with some android devices not working in the background. Check if your device model is on [dontkillmyapp list](https://dontkillmyapp.com) before you report new issue. For more information check out [dontkillmyapp.com](https://dontkillmyapp.com/problem).
Another confusing fact about Android services is concept of foreground services. Foreground service in context of Android OS is different thing than background geolocation service of this plugin (they're related thought). **Plugin's background geolocation service** actually **becomes foreground service** when app is in the background. Confusing, right? :D
If service wants to continue to run in the background, it must "promote" itself to `foreground service`. Foreground services must have visible notification, which is the reason, why you can't disable drawer notification.
The notification can only be disabled, when app is running in the foreground, by setting config option `startForeground: false` (this is the default option), but will always be visible in the background (if service was started).
Recommend you to read https://developer.android.com/about/versions/oreo/background
## Description
React Native fork of [cordova-plugin-background-geolocation](https://github.com/mauron85/cordova-plugin-background-geolocation)
with battery-saving "circular region monitoring" and "stop detection".
This plugin can be used for geolocation when the app is running in the foreground or background.
You can choose from following location providers:
* **DISTANCE_FILTER_PROVIDER**
* **ACTIVITY_PROVIDER**
* **RAW_PROVIDER**
See [Which provider should I use?](/PROVIDERS.md) for more information about providers.
## Dependencies
Versions of libraries and sdk versions used to compile this plugin can be overriden in
`android/build.gradle` with ext declaration.
When ext is not provided then following defaults will be used:
```
ext {
compileSdkVersion = 28
buildToolsVersion = "28.0.3"
targetSdkVersion = 28
minSdkVersion = 16
supportLibVersion = "28.0.0"
googlePlayServicesVersion = "11+"
}
```
## Compatibility
Due to the rapid changes being made in the React Native ecosystem, this module will support
only the latest version of React Native. Older versions will only be supported if they're
compatible with this module.
| Module | React Native |
|------------------|-------------------|
| 0.1.0 - 0.2.0 | 0.33 |
| >=0.3.0 | >=0.47 |
| >=0.6.0 | >=0.60 |
If you are using an older version of React Native with this module some features may be buggy.
If you are using `react-native-maps` or another lib that requires `Google Play Services` such as `Exponent.js`, then in addition to the instalation steps described here, you must set `Google Play Services` library version to match the version used by those libraries. (in this case `9.8.0`)
Add following to `android/build.gradle`
```
ext {
googlePlayServicesVersion = "9.8.0"
}
```
## Example Apps
The repository [react-native-background-geolocation-example](https://github.com/mauron85/react-native-background-geolocation-example) hosts an example app for both iOS and Android platform.
## Quick example
```javascript
import React, { Component } from 'react';
import { Alert } from 'react-native';
import BackgroundGeolocation from '@mauron85/react-native-background-geolocation';
class BgTracking extends Component {
componentDidMount() {
BackgroundGeolocation.configure({
desiredAccuracy: BackgroundGeolocation.HIGH_ACCURACY,
stationaryRadius: 50,
distanceFilter: 50,
notificationTitle: 'Background tracking',
notificationText: 'enabled',
debug: true,
startOnBoot: false,
stopOnTerminate: true,
locationProvider: BackgroundGeolocation.ACTIVITY_PROVIDER,
interval: 10000,
fastestInterval: 5000,
activitiesInterval: 10000,
stopOnStillActivity: false,
url: 'http://192.168.81.15:3000/location',
httpHeaders: {
'X-FOO': 'bar'
},
// customize post properties
postTemplate: {
lat: '@latitude',
lon: '@longitude',
foo: 'bar' // you can also add your own properties
}
});
BackgroundGeolocation.on('location', (location) => {
// handle your locations here
// to perform long running operation on iOS
// you need to create background task
BackgroundGeolocation.startTask(taskKey => {
// execute long running task
// eg. ajax post location
// IMPORTANT: task has to be ended by endTask
BackgroundGeolocation.endTask(taskKey);
});
});
BackgroundGeolocation.on('stationary', (stationaryLocation) => {
// handle stationary locations here
Actions.sendLocation(stationaryLocation);
});
BackgroundGeolocation.on('error', (error) => {
console.log('[ERROR] BackgroundGeolocation error:', error);
});
BackgroundGeolocation.on('start', () => {
console.log('[INFO] BackgroundGeolocation service has been started');
});
BackgroundGeolocation.on('stop', () => {
console.log('[INFO] BackgroundGeolocation service has been stopped');
});
BackgroundGeolocation.on('authorization', (status) => {
console.log('[INFO] BackgroundGeolocation authorization status: ' + status);
if (status !== BackgroundGeolocation.AUTHORIZED) {
// we need to set delay or otherwise alert may not be shown
setTimeout(() =>
Alert.alert('App requires location tracking permission', 'Would you like to open app settings?', [
{ text: 'Yes', onPress: () => BackgroundGeolocation.showAppSettings() },
{ text: 'No', onPress: () => console.log('No Pressed'), style: 'cancel' }
]), 1000);
}
});
BackgroundGeolocation.on('background', () => {
console.log('[INFO] App is in background');
});
BackgroundGeolocation.on('foreground', () => {
console.log('[INFO] App is in foreground');
});
BackgroundGeolocation.on('abort_requested', () => {
console.log('[INFO] Server responded with 285 Updates Not Required');
// Here we can decide whether we want stop the updates or not.
// If you've configured the server to return 285, then it means the server does not require further update.
// So the normal thing to do here would be to `BackgroundGeolocation.stop()`.
// But you might be counting on it to receive location updates in the UI, so you could just reconfigure and set `url` to null.
});
BackgroundGeolocation.on('http_authorization', () => {
console.log('[INFO] App needs to authorize the http requests');
});
BackgroundGeolocation.checkStatus(status => {
console.log('[INFO] BackgroundGeolocation service is running', status.isRunning);
console.log('[INFO] BackgroundGeolocation services enabled', status.locationServicesEnabled);
console.log('[INFO] BackgroundGeolocation auth status: ' + status.authorization);
// you don't need to check status before start (this is just the example)
if (!status.isRunning) {
BackgroundGeolocation.start(); //triggers start on start event
}
});
// you can also just start without checking for status
// BackgroundGeolocation.start();
}
componentWillUnmount() {
// unregister all event listeners
BackgroundGeolocation.removeAllListeners();
}
}
export default BgTracking;
```
## Instalation
### Installation
Add the package to your project
```
yarn add @mauron85/react-native-background-geolocation
```
### Automatic setup
Since version 0.60 React Native does linking of modules [automatically](https://github.com/react-native-community/cli/blob/master/docs/autolinking.md). However it does it only for single module.
As plugin depends on additional 'common' module, it is required to link it with:
```
node ./node_modules/@mauron85/react-native-background-geolocation/scripts/postlink.js
```
### Manual setup
#### Android setup
In `android/settings.gradle`
```gradle
...
include ':@mauron85_react-native-background-geolocation-common'
project(':@mauron85_react-native-background-geolocation-common').projectDir = new File(rootProject.projectDir, '../node_modules/@mauron85/react-native-background-geolocation/android/common')
include ':@mauron85_react-native-background-geolocation'
project(':@mauron85_react-native-background-geolocation').projectDir = new File(rootProject.projectDir, '../node_modules/@mauron85/react-native-background-geolocation/android/lib')
...
```
In `android/app/build.gradle`
```gradle
dependencies {
...
compile project(':@mauron85_react-native-background-geolocation')
...
}
```
Register the module (in `MainApplication.java`)
```java
import com.marianhello.bgloc.react.BackgroundGeolocationPackage; // <--- Import Package
public class MainApplication extends Application implements ReactApplication {
...
/**
* A list of packages used by the app. If the app uses additional views
* or modules besides the default ones, add more packages here.
*/
@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(),
new BackgroundGeolocationPackage() // <---- Add the Package
);
}
...
}
```
#### iOS setup
1. In XCode, in the project navigator, right click `Libraries` ➜ `Add Files to [your project's name]`
2. Add `./node_modules/@mauron85/react-native-background-geolocation/ios/RCTBackgroundGeolocation.xcodeproj`
3. In the XCode project navigator, select your project, select the `Build Phases` tab and in the `Link Binary With Libraries` section add **libRCTBackgroundGeolocation.a**
4. Add `UIBackgroundModes` **location** to `Info.plist`
5. Add `NSMotionUsageDescription` **App requires motion tracking** to `Info.plist` (required by ACTIVITY_PROVIDER)
For iOS before version 11:
6. Add `NSLocationAlwaysUsageDescription` **App requires background tracking** to `Info.plist`
For iOS 11:
6. Add `NSLocationWhenInUseUsageDescription` **App requires background tracking** to `Info.plist`
7. Add `NSLocationAlwaysAndWhenInUseUsageDescription` **App requires background tracking** to `Info.plist`
## API
### configure(options, success, fail)
| Parameter | Type | Platform | Description |
|-----------|---------------|----------|---------------------------------------------------------------------------------|
| `options` | `JSON Object` | all | Configure options |
Configure options:
| Parameter | Type | Platform | Description | Provider* | Default |
|---------------------------|-------------------|--------------|----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|-------------|----------------------------|
| `locationProvider` | `Number` | all | Set location provider **@see** [PROVIDERS](/PROVIDERS.md) | N/A | DISTANCE\_FILTER\_PROVIDER |
| `desiredAccuracy` | `Number` | all | Desired accuracy in meters. Possible values [HIGH_ACCURACY, MEDIUM_ACCURACY, LOW_ACCURACY, PASSIVE_ACCURACY]. Accuracy has direct effect on power drain. Lower accuracy = lower power drain. | all | MEDIUM\_ACCURACY |
| `stationaryRadius` | `Number` | all | Stationary radius in meters. When stopped, the minimum distance the device must move beyond the stationary location for aggressive background-tracking to engage. | DIS | 50 |
| `debug` | `Boolean` | all | When enabled, the plugin will emit sounds for life-cycle events of background-geolocation! See debugging sounds table. | all | false |
| `distanceFilter` | `Number` | all | The minimum distance (measured in meters) a device must move horizontally before an update event is generated. **@see** [Apple docs](https://developer.apple.com/library/ios/documentation/CoreLocation/Reference/CLLocationManager_Class/CLLocationManager/CLLocationManager.html#//apple_ref/occ/instp/CLLocationManager/distanceFilter). | DIS,RAW | 500 |
| `stopOnTerminate` | `Boolean` | all | Enable this in order to force a stop() when the application terminated (e.g. on iOS, double-tap home button, swipe away the app). | all | true |
| `startOnBoot` | `Boolean` | Android | Start background service on device boot. | all | false |
| `interval` | `Number` | Android | The minimum time interval between location updates in milliseconds. **@see** [Android docs](http://developer.android.com/reference/android/location/LocationManager.html#requestLocationUpdates(long,%20float,%20android.location.Criteria,%20android.app.PendingIntent)) for more information. | all | 60000 |
| `fastestInterval` | `Number` | Android | Fastest rate in milliseconds at which your app can handle location updates. **@see** [Android docs](https://developers.google.com/android/reference/com/google/android/gms/location/LocationRequest.html#getFastestInterval()). | ACT | 120000 |
| `activitiesInterval` | `Number` | Android | Rate in milliseconds at which activity recognition occurs. Larger values will result in fewer activity detections while improving battery life. | ACT | 10000 |
| `stopOnStillActivity` | `Boolean` | Android | @deprecated stop location updates, when the STILL activity is detected | ACT | true |
| `notificationsEnabled` | `Boolean` | Android | Enable/disable local notifications when tracking and syncing locations | all | true |
| `startForeground` | `Boolean` | Android | Allow location sync service to run in foreground state. Foreground state also requires a notification to be presented to the user. | all | false |
| `notificationTitle` | `String` optional | Android | Custom notification title in the drawer. (goes with `startForeground`) | all | "Background tracking" |
| `notificationText` | `String` optional | Android | Custom notification text in the drawer. (goes with `startForeground`) | all | "ENABLED" |
| `notificationIconColor` | `String` optional | Android | The accent color to use for notification. Eg. **#4CAF50**. (goes with `startForeground`) | all | |
| `notificationIconLarge` | `String` optional | Android | The filename of a custom notification icon. **@see** Android quirks. (goes with `startForeground`) | all | |
| `notificationIconSmall` | `String` optional | Android | The filename of a custom notification icon. **@see** Android quirks. (goes with `startForeground`) | all | |
| `activityType` | `String` | iOS | [AutomotiveNavigation, OtherNavigation, Fitness, Other] Presumably, this affects iOS GPS algorithm. **@see** [Apple docs](https://developer.apple.com/library/ios/documentation/CoreLocation/Reference/CLLocationManager_Class/CLLocationManager/CLLocationManager.html#//apple_ref/occ/instp/CLLocationManager/activityType) for more information | all | "OtherNavigation" |
| `pauseLocationUpdates` | `Boolean` | iOS | Pauses location updates when app is paused. **@see* [Apple docs](https://developer.apple.com/documentation/corelocation/cllocationmanager/1620553-pauseslocationupdatesautomatical?language=objc) | all | false |
| `saveBatteryOnBackground` | `Boolean` | iOS | Switch to less accurate significant changes and region monitory when in background | all | false |
| `url` | `String` | all | Server url where to send HTTP POST with recorded locations **@see** [HTTP locations posting](#http-locations-posting) | all | |
| `syncUrl` | `String` | all | Server url where to send fail to post locations **@see** [HTTP locations posting](#http-locations-posting) | all | |
| `syncThreshold` | `Number` | all | Specifies how many previously failed locations will be sent to server at once | all | 100 |
| `httpHeaders` | `Object` | all | Optional HTTP headers sent along in HTTP request | all | |
| `maxLocations` | `Number` | all | Limit maximum number of locations stored into db | all | 10000 |
| `postTemplate` | `Object\|Array` | all | Customization post template **@see** [Custom post template](#custom-post-template) | all | |
\*
DIS = DISTANCE\_FILTER\_PROVIDER
ACT = ACTIVITY\_PROVIDER
RAW = RAW\_PROVIDER
Partial reconfiguration is possible by later providing a subset of the configuration options:
```
BackgroundGeolocation.configure({
debug: true
});
```
In this case new configuration options will be merged with stored configuration options and changes will be applied immediately.
**Important:** Because configuration options are applied partially, it's not possible to reset option to default value just by omitting it's key name and calling `configure` method. To reset configuration option to the default value, it's key must be set to `null`!
```
// Example: reset postTemplate to default
BackgroundGeolocation.configure({
postTemplate: null
});
```
### getConfig(success, fail)
Platform: iOS, Android
Get current configuration. Method will return all configuration options and their values in success callback.
Because `configure` method can be called with subset of the configuration options only,
`getConfig` method can be used to check the actual applied configuration.
```
BackgroundGeolocation.getConfig(function(config) {
console.log(config);
});
```
### start()
Platform: iOS, Android
Start background geolocation.
### stop()
Platform: iOS, Android
Stop background geolocation.
### getCurrentLocation(success, fail, options)
Platform: iOS, Android
One time location check to get current location of the device.
| Option parameter | Type | Description |
|----------------------------|-----------|----------------------------------------------------------------------------------------|
| `timeout` | `Number` | Maximum time in milliseconds device will wait for location |
| `maximumAge` | `Number` | Maximum age in milliseconds of a possible cached location that is acceptable to return |
| `enableHighAccuracy` | `Boolean` | if true and if the device is able to provide a more accurate position, it will do so |
| Success callback parameter | Type | Description |
|----------------------------|-----------|----------------------------------------------------------------|
| `location` | `Object` | location object (@see [Location event](#location-event)) |
| Error callback parameter | Type | Description |
|----------------------------|-----------|----------------------------------------------------------------|
| `code` | `Number` | Reason of an error occurring when using the geolocating device |
| `message` | `String` | Message describing the details of the error |
Error codes:
| Value | Associated constant | Description |
|-------|----------------------|--------------------------------------------------------------------------|
| 1 | PERMISSION_DENIED | Request failed due missing permissions |
| 2 | LOCATION_UNAVAILABLE | Internal source of location returned an internal error |
| 3 | TIMEOUT | Timeout defined by `option.timeout was exceeded |
### checkStatus(success, fail)
Check status of the service
| Success callback parameter | Type | Description |
|----------------------------|-----------|------------------------------------------------------|
| `isRunning` | `Boolean` | true/false (true if service is running) |
| `locationServicesEnabled` | `Boolean` | true/false (true if location services are enabled) |
| `authorization` | `Number` | authorization status |
Authorization statuses:
* NOT_AUTHORIZED
* AUTHORIZED - authorization to run in background and foreground
* AUTHORIZED_FOREGROUND iOS only authorization to run in foreground only
Note: In the Android concept of authorization, these represent application permissions.
### showAppSettings()
Platform: Android >= 6, iOS >= 8.0
Show app settings to allow change of app location permissions.
### showLocationSettings()
Platform: Android
Show system settings to allow configuration of current location sources.
### getLocations(success, fail)
Platform: iOS, Android
Method will return all stored locations.
This method is useful for initial rendering of user location on a map just after application launch.
| Success callback parameter | Type | Description |
|----------------------------|---------|--------------------------------|
| `locations` | `Array` | collection of stored locations |
```javascript
BackgroundGeolocation.getLocations(
function (locations) {
console.log(locations);
}
);
```
### getValidLocations(success, fail)
Platform: iOS, Android
Method will return locations which have not yet been posted to server.
| Success callback parameter | Type | Description |
|----------------------------|---------|--------------------------------|
| `locations` | `Array` | collection of stored locations |
### deleteLocation(locationId, success, fail)
Platform: iOS, Android
Delete location with locationId.
### deleteAllLocations(success, fail)
Note: You don't need to delete all locations. The plugin manages the number of stored locations automatically and the total count never exceeds the number as defined by `option.maxLocations`.
Platform: iOS, Android
Delete all stored locations.
Note: Locations are not actually deleted from database to avoid gaps in locationId numbering.
Instead locations are marked as deleted. Locations marked as deleted will not appear in output of `BackgroundGeolocation.getValidLocations`.
### switchMode(modeId, success, fail)
Platform: iOS
Normally the plugin will handle switching between **BACKGROUND** and **FOREGROUND** mode itself.
Calling switchMode you can override plugin behavior and force it to switch into other mode.
In **FOREGROUND** mode the plugin uses iOS local manager to receive locations and behavior is affected
by `option.desiredAccuracy` and `option.distanceFilter`.
In **BACKGROUND** mode plugin uses significant changes and region monitoring to receive locations
and uses `option.stationaryRadius` only.
```
// switch to FOREGROUND mode
BackgroundGeolocation.switchMode(BackgroundGeolocation.FOREGROUND_MODE);
// switch to BACKGROUND mode
BackgroundGeolocation.switchMode(BackgroundGeolocation.BACKGROUND_MODE);
```
### forceSync()
Platform: Android, iOS
Force sync of pending locations. Option `syncThreshold` will be ignored and
all pending locations will be immediately posted to `syncUrl` in single batch.
### getLogEntries(limit, fromId, minLevel, success, fail)
Platform: Android, iOS
Return all logged events. Useful for plugin debugging.
| Parameter | Type | Description |
|------------|---------------|---------------------------------------------------------------------------------------------------|
| `limit` | `Number` | limits number of returned entries |
| `fromId` | `Number` | return entries after fromId. Useful if you plan to implement infinite log scrolling* |
| `minLevel` | `String` | return log entries above level. Available levels: ["TRACE", "DEBUG", "INFO", "WARN", "ERROR] |
| `success` | `Function` | callback function which will be called with log entries |
*[Example of infinite log scrolling](https://github.com/mauron85/react-native-background-geolocation-example/blob/master/src/scenes/Logs.js)
Format of log entry:
| Parameter | Type | Description |
|-------------|---------------|---------------------------------------------------------------------------------------------------|
| `id` | `Number` | id of log entry as stored in db |
| `timestamp` | `Number` | timestamp in milliseconds since beginning of UNIX epoch |
| `level` | `String` | log level |
| `message` | `String` | log message |
| `stackTrace`| `String` | recorded stacktrace (Android only, on iOS part of message) |
### removeAllListeners(event)
Unregister all event listeners for given event. If parameter `event` is not provided then all event listeners will be removed.
## Events
| Name | Callback param | Platform | Provider* | Description |
|---------------------|------------------------|--------------|-------------|--------------------------------------------------|
| `location` | `Location` | all | all | on location update |
| `stationary` | `Location` | all | DIS,ACT | on device entered stationary mode |
| `activity` | `Activity` | Android | ACT | on activity detection |
| `error` | `{ code, message }` | all | all | on plugin error |
| `authorization` | `status` | all | all | on user toggle location service |
| `start` | | all | all | geolocation has been started |
| `stop` | | all | all | geolocation has been stopped |
| `foreground` | | Android | all | app entered foreground state (visible) |
| `background` | | Android | all | app entered background state |
| `abort_requested` | | all | all | server responded with "285 Updates Not Required" |
| `http_authorization`| | all | all | server responded with "401 Unauthorized" |
### Location event
| Location parameter | Type | Description |
|------------------------|-----------|------------------------------------------------------------------------|
| `id` | `Number` | ID of location as stored in DB (or null) |
| `provider` | `String` | gps, network, passive or fused |
| `locationProvider` | `Number` | location provider |
| `time` | `Number` | UTC time of this fix, in milliseconds since January 1, 1970. |
| `latitude` | `Number` | Latitude, in degrees. |
| `longitude` | `Number` | Longitude, in degrees. |
| `accuracy` | `Number` | Estimated accuracy of this location, in meters. |
| `speed` | `Number` | Speed if it is available, in meters/second over ground. |
| `altitude` | `Number` | Altitude if available, in meters above the WGS 84 reference ellipsoid. |
| `bearing` | `Number` | Bearing, in degrees. |
| `isFromMockProvider` | `Boolean` | (android only) True if location was recorded by mock provider |
| `mockLocationsEnabled` | `Boolean` | (android only) True if device has mock locations enabled |
Locations parameters `isFromMockProvider` and `mockLocationsEnabled` are not posted to `url` or `syncUrl` by default.
Both can be requested via option `postTemplate`.
Note: Do not use location `id` as unique key in your database as ids will be reused when `option.maxLocations` is reached.
Note: Android currently returns `time` as type of String (instead of Number) [@see issue #9685](https://github.com/facebook/react-native/issues/9685)
### Activity event
| Activity parameter | Type | Description |
|--------------------|-----------|------------------------------------------------------------------------|
| `confidence` | `Number` | Percentage indicating the likelihood user is performing this activity. |
| `type` | `String` | "IN_VEHICLE", "ON_BICYCLE", "ON_FOOT", "RUNNING", "STILL", |
| | | "TILTING", "UNKNOWN", "WALKING" |
Event listeners can registered with:
```
const eventSubscription = BackgroundGeolocation.on('event', callbackFn);
```
And unregistered:
```
eventSubscription.remove();
```
Note: Components should unregister all event listeners in `componentWillUnmount` method,
individually, or with `removeAllListeners`
## HTTP locations posting
All locations updates are recorded in the local db at all times. When the App is in foreground or background, in addition to storing location in local db,
the location callback function is triggered. The number of locations stored in db is limited by `option.maxLocations` and never exceeds this number.
Instead, old locations are replaced by new ones.
When `option.url` is defined, each location is also immediately posted to url defined by `option.url`.
If the post is successful, the location is marked as deleted in local db.
When `option.syncUrl` is defined, all locations that fail to post will be coalesced and sent in some time later in a single batch.
Batch sync takes place only when the number of failed-to-post locations reaches `option.syncTreshold`.
Locations are sent only in single batch when the number of locations reaches `option.syncTreshold`. (No individual locations will be sent)
The request body of posted locations is always an array, even when only one location is sent.
Warning: `option.maxLocations` has to be larger than `option.syncThreshold`. It's recommended to be 2x larger. In any other case the location syncing might not work properly.
## Custom post template
With `option.postTemplate` it is possible to specify which location properties should be posted to `option.url` or `option.syncUrl`. This can be useful to reduce the
number of bytes sent "over the "wire".
All wanted location properties have to be prefixed with `@`. For all available properties check [Location event](#location-event).
Two forms are supported:
**jsonObject**
```
BackgroundGeolocation.configure({
postTemplate: {
lat: '@latitude',
lon: '@longitude',
foo: 'bar' // you can also add your own properties
}
});
```
**jsonArray**
```
BackgroundGeolocation.configure({
postTemplate: ['@latitude', '@longitude', 'foo', 'bar']
});
```
Note: Keep in mind that all locations (even a single one) will be sent as an array of object(s), when postTemplate is `jsonObject` and array of array(s) for `jsonArray`!
### Android Headless Task (Experimental)
A special task that gets executed when the app is terminated, but the plugin was configured to continue running in the background (option `stopOnTerminate: false`). In this scenario the [Activity](https://developer.android.com/reference/android/app/Activity.html)
was killed by the system and all registered event listeners will not be triggered until the app is relaunched.
**Note:** Prefer configuration options `url` and `syncUrl` over headless task. Use it sparingly!
#### Task event
| Parameter | Type | Description |
|--------------------|-----------|------------------------------------------------------------------------|
| `event.name` | `String` | Name of the event [ "location", "stationary", "activity" ] |
| `event.params` | `Object` | Event parameters. @see [Events](#events) |
Keep in mind that the callback function lives in an isolated scope. Variables from a higher scope cannot be referenced!
Following example requires [CORS](https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS) enabled backend server.
**Warning:** callback function must by `async`!
```
BackgroundGeolocation.headlessTask(async (event) => {
if (event.name === 'location' ||
event.name === 'stationary') {
var xhr = new XMLHttpRequest();
xhr.open('POST', 'http://192.168.81.14:3000/headless');
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.send(JSON.stringify(event.params));
}
});
```
**Important:**
After application is launched again (main activity becomes visible), it is important to call `start` method to rebind all event listeners.
```
BackgroundGeolocation.checkStatus(({ isRunning }) => {
if (isRunning) {
BackgroundGeolocation.start(); // service was running -> rebind all listeners
}
});
```
### Transforming/filtering locations in native code
In some cases you might want to modify a location before posting, reject a location, or any other logic around incoming locations - in native code. There's an option of doing so with a headless task, but you may want to preserve battery, or do more complex actions that are not available in React.
In those cases you could register a location transform.
Android example:
When the `Application` is initialized (which also happens before services gets started in the background), write some code like this:
```
BackgroundGeolocationFacade.setLocationTransform(new LocationTransform() {
@Nullable
@Override
public BackgroundLocation transformLocationBeforeCommit(@NonNull Context context, @NonNull BackgroundLocation location) {
// `context` is available too if there's a need to use a value from preferences etc.
// Modify the location
location.setLatitude(location.getLatitude() + 0.018);
// Return modified location
return location;
// You could return null to reject the location,
// or if you did something else with the location and the library should not post or save it.
}
});
```
iOS example:
In `didFinishLaunchingWithOptions` delegate method, write some code like this:
```
BackgroundGeolocationFacade.locationTransform = ^(MAURLocation * location) {
// Modify the location
location.latitude = @(location.latitude.doubleValue + 0.018);
// Return modified location
return location;
// You could return null to reject the location,
// or if you did something else with the location and the library should not post or save it.
};
```
### Advanced plugin configuration
#### Change Account Service Name (Android)
Add string resource "account_name" into "android/app/src/main/res/values/strings.xml"
```
<string name="account_name">Sync Locations</string>
```
### Example of backend server
[Background-geolocation-server](https://github.com/mauron85/background-geolocation-server) is a backend server written in nodejs with CORS - Cross-Origin Resource Sharing support.
There are instructions how to run it and simulate locations on Android, iOS Simulator and Genymotion.
## Debugging
## Submit crash log
TODO
## Debugging sounds
| Event | *ios* | *android* |
|-------------------------------------|-----------------------------------|-------------------------|
| Exit stationary region | Calendar event notification sound | dialtone beep-beep-beep |
| Geolocation recorded | SMS sent sound | tt short beep |
| Aggressive geolocation engaged | SIRI listening sound | |
| Passive geolocation engaged | SIRI stop listening sound | |
| Acquiring stationary location sound | "tick,tick,tick" sound | |
| Stationary location acquired sound | "bloom" sound | long tt beep |
**NOTE:** For iOS in addition, you must manually enable the *Audio and Airplay* background mode in *Background Capabilities* to hear these debugging sounds.
## Geofencing
Try using [react-native-boundary](https://github.com/eddieowens/react-native-boundary). Let's keep this plugin lightweight as much as possible.
## Changelog
See [CHANGES.md](/CHANGES.md)
See [变更(非官方中文版)](/CHANGES_zh-Hans.md)
| 0 |
renrenio/renren-security | 采用Spring、MyBatis、Shiro框架,开发的一套权限系统,极低门槛,拿来即用。设计之初,就非常注重安全性,为企业系统保驾护航,让一切都变得如此简单。【QQ群:324780204、145799952】 | null | ### 项目说明
- renren-security是一个轻量级的,前后端分离的Java快速开发平台,能快速开发项目并交付【接私活利器】
- 采用SpringBoot、Shiro、MyBatis-Plus框架,开发的一套权限系统,极低门槛,拿来即用。设计之初,就非常注重安全性,为企业系统保驾护航,让一切都变得如此简单。
- 提供了代码生成器,只需编写30%左右代码,其余的代码交给系统自动生成,可快速完成开发任务
- 支持MySQL、Oracle、SQL Server、PostgreSQL等主流数据库
- 前端地址:https://gitee.com/renrenio/renren-ui
- 演示地址:http://demo.open.renren.io/renren-security (账号密码:admin/admin)
<br>
### 微信交流群
我们提供了微信交流群,扫码下面的二维码,关注【人人开源】公众号,回复【加群】,即可根据提示加入微信群!
<br><br>

<br>
### 具有如下特点
- 友好的代码结构及注释,便于阅读及二次开发
- 实现前后端分离,通过token进行数据交互,前端再也不用关注后端技术
- 灵活的权限控制,可控制到页面或按钮,满足绝大部分的权限需求
- 提供CrudService接口,对增删改查进行封装,代码更简洁
- 页面交互使用Vue2.x,极大的提高了开发效率
- 完善的部门管理及数据权限,通过注解实现数据权限的控制
- 完善的XSS防范及脚本过滤,彻底杜绝XSS攻击
- 完善的代码生成机制,可在线生成entity、xml、dao、service、vue、sql代码,减少70%以上的开发任务
- 引入quartz定时任务,可动态完成任务的添加、修改、删除、暂停、恢复及日志查看等功能
- 引入Hibernate Validator校验框架,轻松实现后端校验
- 引入云存储服务,已支持:七牛云、阿里云、腾讯云等
- 引入swagger文档支持,方便编写API接口文档
<br>
### 数据权限设计思想
- 用户管理、角色管理、部门管理,可操作本部门及子部门数据
- 菜单管理、定时任务、参数管理、字典管理、系统日志,没有数据权限
- 业务功能,按照用户数据权限,查询、操作数据【没有本部门数据权限,也能查询本人数据】
<br>
**项目结构**
```
renren-security
├─renren-common 公共模块
│
├─renren-admin 管理后台
│ ├─db 数据库SQL脚本
│ │
│ ├─modules 模块
│ │ ├─job 定时任务
│ │ ├─log 日志管理
│ │ ├─oss 文件存储
│ │ ├─security 安全模块
│ │ └─sys 系统管理(核心)
│ │
│ └─resources
│ ├─mapper MyBatis文件
│ ├─public 静态资源
│ └─application.yml 全局配置文件
│
│
├─renren-api API服务
│
├─renren-generator 代码生成器
│ └─resources
│ ├─mapper MyBatis文件
│ ├─template 代码生成器模板(可增加或修改相应模板)
│ ├─application.yml 全局配置文件
│ └─generator.properties 代码生成器,配置文件
│
```
<br>
**技术选型:**
- 核心框架:Spring Boot 2.6
- 安全框架:Apache Shiro 1.9
- 持久层框架:MyBatis 3.5
- 定时器:Quartz 2.3
- 数据库连接池:Druid 1.2
- 日志管理:Logback
- 页面交互:Vue2.x
<br>
**软件需求**
- JDK1.8
- Maven3.0+
- MySQL8.0
- Oracle 11g+
- SQL Server 2012+
- PostgreSQL 9.4+
<br>
**本地部署**
- 通过git下载源码
- idea、eclipse需安装lombok插件,不然会提示找不到entity的get set方法
- 创建数据库renren_security,数据库编码为UTF-8
- 执行db/mysql.sql文件,初始化数据
- 修改application-dev.yml文件,更新MySQL账号和密码
- 在renren-security目录下,执行mvn clean install
- Eclipse、IDEA运行AdminApplication.java,则可启动项目【renren-admin】
- renren-admin访问路径:http://localhost:8080/renren-admin
- swagger文档路径:http://localhost:8080/renren-admin/doc.html
- 再启动前端项目,前端地址:https://gitee.com/renrenio/renren-ui
- 账号密码:admin/admin
<br>

<br>

<br>
### 如何交流、反馈、参与贡献?
- 开发文档:https://www.renren.io/guide/security
- 官方社区:https://www.renren.io/community
- Gitee仓库:https://gitee.com/renrenio/renren-security
- [人人开源](https://www.renren.io):https://www.renren.io
- 如需关注项目最新动态,请Watch、Star项目,同时也是对项目最好的支持
- 技术讨论、二次开发等咨询、问题和建议,请移步到官方社区,我会在第一时间进行解答和回复!
<br>
| 0 |
microsoft/gctoolkit | Tool for parsing GC logs | gc heap java jvm memory memory-management performance | # Microsoft GCToolKit
GCToolkit is a set of libraries for analyzing HotSpot Java garbage collection (GC) log files. The toolkit parses GC log files into discrete events and provides an API for aggregating data from those events. This allows the user to create arbitrary and complex analyses of the state of managed memory in the Java Virtual Machine (JVM) represented by the garbage collection log.
For more detail you can read our [Launch Blog Post](https://devblogs.microsoft.com/java/introducing-microsoft-gctoolkit/).
[](https://github.com/microsoft/gctoolkit/actions/workflows/maven.yml)
---
## Introduction
Managed memory in the Java Virtual Machine (JVM) is composed of 3 main pieces:
1. Memory buffers known as Java heap
1. Allocators which perform the work of getting data into Java heap
1. Garbage Collection (GC).
While GC is responsible for recovering memory in Java heap that is no longer in use, the term is often used as a euphemism for memory management. The phrasing of _Tuning GC_ or _tuning the collector_ are often used with the understanding that it refers to tuning the JVM’s memory management subsystem. The best source of telemetry data for tuning GC comes from GC Logs and GCToolKit has been helpful in making this task easier by providing parsers, models and an API to build analytics with. You can run the Maven project [HeapOccupancyAfterCollectionSummary sample](./sample/README.md) as an example of this.
## Usage
In order to use this library you'll need to add its dependencies to your project. We provide the instructions for Maven below.
### Maven Coordinates
[](https://search.maven.org/search?q=g:%22com.microsoft.gctoolkit%22%20AND%20a:%22gctoolkit-api%22)
The GCToolKit artifacts are in [Maven Central](https://search.maven.org/search?q=g:com.microsoft.gctoolkit). You'll then need to add the `api`, `parser` and `vertx` modules to your project in the `dependencyManagement` and/or `dependencies` section as you see fit.
```xml
<dependencies>
...
<dependency>
<groupId>com.microsoft.gctoolkit</groupId>
<artifactId>api</artifactId>
<version>3.0.4</version>
</dependency>
<dependency>
<groupId>com.microsoft.gctoolkit</groupId>
<artifactId>parser</artifactId>
<version>3.0.4</version>
</dependency>
<dependency>
<groupId>com.microsoft.gctoolkit</groupId>
<artifactId>vertx</artifactId>
<version>3.0.4</version>
</dependency>
...
</dependencies>
```
## User Discussions
Meet other developers working with GCToolKit, ask questions, and participate in the development of this project by visiting the [Discussions](https://github.com/microsoft/gctoolkit/discussions) tab.
### Example
See the sample project: [sample/README](./sample/README.md)
## Contributing
See [CONTRIBUTING](CONTRIBUTING.md) for full details including more options for building and testing the project.
### Test Coverage Report
**Core API Coverage** </br>
**Core :: Parser</br>**
**Core :: Vertx**</br>
## License
Microsoft GCToolKit is licensed under the [MIT](https://github.com/microsoft/gctoolkit/blob/master/LICENSE) license.
| 0 |
201206030/novel-cloud | novel-cloud 是基于 novel 构建的 Spring Cloud 微服务技术栈学习型小说项目。选用了 Spring Boot 3 、Spring Cloud 2022、Spring Cloud Alibaba 2022、MyBatis-Plus、ShardingSphere-JDBC、Redis、RabbitMQ、Elasticsearch 、XXL-JOB 等流行技术。 | microsoft-services spring-boot spring-cloud spring-cloud-alibaba study-project | []( https://cloud.tencent.com/act/cps/redirect?redirect=2446&cps_key=736e609d66e0ac4e57813316cec6fd0b&from=console )
<p align="center">
<a href='https://docs.oracle.com/en/java/javase/17'><img alt="Java 17" src="https://img.shields.io/badge/Java%2017-%234479A1.svg?logo=data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAMgAAADICAMAAACahl6sAAABNVBMVEUAAABkmP9ml/9mmf9mmf9lmv9nmf9mmf9mmf9nmP9mmf9mmf9mmv9mmf9mmf9mmf9mmf9mmP9llv9mmf9mmf9mmv9mmf9mmf9mmf9mmf//AABlmf9mmf9km/9mmf9mmf9lmf9mmf9mmf//AABmmf9mmf9mmf9lmv9mmf//AABmmf9mmP9mmf9mmf//AABgl/9mmf//AABmmP//AABmmf//AAD/AABmmf9mmv9mmf//AABnmf//AAD/AAD/AABmmf//AAD/AABlmf9mmf//AABmmf9mmv9mmf//AAD/AAD/AABmmf//AAD/AAD/AAD/AAD/AAD/AAD/AAD/AAD/AABmmf//AAD/AAD/AABsof9mmf//AAD/AAD/AAD/AAD/AAD/AAD/AAD/AAD/AAD/AABmmf//AAB37HanAAAAZXRSTlMAP4CLnb8dtpUP2plK78zEpyoKrGbVbxj334BjRzL7sVA168C8hXlEIwvJYJBXQQahWC8k5M6nW1Q6Myb2ya5yY0gT0rp9dWpSEerm35JtH5l7Xhjnzo2EdATz2dOfiDst8AW1KD5Fo/kAAAl3SURBVHja3Ny7ruIwEAbg/zloU9FEiqJQoCQiEsUh4k4ESNw56H//R1gHlsQk8TnbrcdfS8OIsT2escB/MoEjlnDEfAQ3ZMUDTlgwhRvIIZyQcAcneDzc4QKPDOACj3TjLPHICC7wSMIFR5JOFFxUXDjcQyoXyJeRbpzteyoLyOdR6UO8kMoN8u2pjCGfR+UM8b5IOlE0jlkKId2aigt33YQl+X2UPUu3DYTzqThwrZokLMVXCLfk0xbC9fiUSy8Ye3zpQbYRXyLhha9P0oXqpIpjANGqOGLZd1yfdGKlB3QjsVZ8SyDZiW+55GnCxmMlxE/sPl/8gpULzL52X7DZiDUfBv0g5dnu3+P8exyT9SDmt92t4E36axzDWU4Wc1jtEv0WR5BSOVneUlnffo5jsTpSiexe5MCOmhBN/jKmYvsix2TAWjFBw/D1se2LHBgmrI3v+LQ582UPy2UFa0s0zKd8Omaw3IiabTPIMV8G1nfpVtT08GEx418r2G5Lzbw7q3iwPq1woiaAblJ9ltrf2vpmLQ+huySC7rsD1o74MLrxJbb9LFfmrHndfUbmPqy3/oc4pgKuu196HHfoepLaD48pK8nG8HvAfvcxK8W1ddTLGfHMWFtDd81JihmCBub6KpXUZ+xHrIwNtVcuIbF6rA2hu7Ik5gFKwsqp684r5dXcwtho6E+piHnaFLCStm9ZgqbSO9PWizHfBNSKevUe9xs5JysQz5RZGUkpPZPPQALjtnyCAN+mPbZHWXO3Hd8WxkBiCQ/7A7712/WJpDcPG77dW8eIrNl0tdo3rV1L1gZcrYWHuXZhJGCVLG6G0W1EUlSVMjMce1sKS65hdxsIGaUl18AwZjvwRcxL2cwwL5jxwxrWW/Jp2siex426qeXDaGUSdS/3FUlZx6LPp0O/3WDRWf7OobTv/qYZPxwtH63XO1dkmCrKGR8iPHQnT0rdAfbLYipF2AxwSp39k1Bg1H2rDaRtXMCuu6Y6U3OEBMvul/xj1nKIkHY2rK8FKzFk8Kj4HatH0gCuukwl7eSS1RiqSvcZGkYSX/oXHd2fkCVp/5Nw6yjYj+IyqxS3z72ZoEbdn3bu/HVpMI4D+Gf37S63uXRebSoeKCKoGQl+UyMpouiEIIhP//+fULoOc1/LdFsuev0ssrfu+Tx7ru15HHnmevUpmyeO7x6OTN6HvWH2Xony5OA9Wh+zMmiPuHP/RaSxP4Eserp/2fcys7R7i+d7Jfh1Zv+PrUd7cxCZ6tIj7n1vMNnO8SPQ/cdZrFdRD+9mYSvK7716kIHZrBM8ewOn8myKNn0p78EV+vACTmKQFQW/q6yHkBZ9QvKabxXaVFWAizVzBWYQ8JU6fiMJkDi9Q1u4xdkexGJCEm9hpyrhV7kmJEm3NRd3mJUOMRFgj0fjVxNICkGqGFIbQ0hMNdkkNUrEkELWIFEr/IqAuBkdDr+hdUgaiSEO4uVRLn7DlSBOswnpQES/jaE5xIks4HddiIs+DiruhnR+2UwsiI/Txj02XKqvV+c8J+IWz8IRGwzNYr1f962GcB5Bl/PBgBnhd0wJjhpgqAix4fHAhiNLApzCcGa1ZrVjL/nKjYIH6vNTfj4S4pPDWyiiakrkukfsaVZ7RbtBUvzA5JiXYr2ARyk+NYO0g0A+h3Gy6GLtLYTSCxKazjmMgctRnZoBpzCTqL8hLy/l8CwFV9UoexJGOJGVWN8ecjy5+KXptt0y/pZi+XSQlz0H/pyOobIASVsMm/JkXFyvlgHF01qFYziN7gYNu9iTiZrOCn24QIAhFbLNEDE0hmyzMVSBbGttIk09YQIkQsJQAOmQIRkTDHUhDbWgA8mYpFixFnMGSUiGjl9B8joWIgMJwZALiZtp+AUPiWhhyIfk+bj1Es5jEHMDjpIxJEHyKAyRLfhDrR7lK4jos7/pCN01pIDBr95JHQ9O05+upXYZv3nZhNs4NO50WUgDg3sKucqgS86LVcJrCZHaphPVfIMacBYe4lmIqIYfqxCQjhIeVX6n1EeuaL1sq6pYx1+pSwdR3lK4pRYhNaSCMVB8aj+KrOIXZhXS1OpeGqKyai5gz1DbtbkppE0nLTyTqNnDw4rMIyITVsH0TXi1gH9mZDaaAkTYCmrFFvxFfS/Pq8pJEXzJlh24FUk338I1YEur7oBru4eJynUx55t0l+x5BhznwPUxFuxsWCOmwxnrCH3477//dmT4F3gVFv4BXeThHzB+iSJk39RERAYyz8Md0oDTOQRcHUPFkEtNT8jSkle0qiDSLbgyxE/DXXpVrBK604dDwrBUJLWbMn5TJq+syhF4m3duzucq2kDiqSAYMGIBo5TuEK6Jieejp3A9HLyEWYKrwb7EsyldHa4IKZ6XYnB1T2f9jo9/SKTHBlyjaXCDJ+PsGlyDxWzKQlSJ4jb4OyOOkv/+4LdPLBlrU/5FvWFlcqCO8BYFkeHzUwEi0p6OEJpLroBflP1GpzRc/DLwrCn3Ouv5kgwV5S+fP0OHgLiVpDpuuVKqbVQbQJxmqzbuMHkBUtXDBsSmqZVxh25C2tZ4AzFheQypMqROt2IL0nH/4jYjwsK4bi0av2lC6sj41r67+B0J6SJ2JwlsiEUH91hrFlIyLPJt/ELyYI8Q25jp3aAKSRPkgNsgRmPAFM5H4aFRZUlAMgxdtmkLv8o19IMeWYbzzRi8RZmhGz1PgLiwRIfU1M1PK9YlOFDNvYVLkBYeU29rQYdw4DyOTkzyy67mi2X8mX/LfvsFhV24zNsxV8ejwnW1NqfxwXbPw5AV4FBfWLAzvTYNt9TOG4Fkqtaxr8xRX0JE5UVUDbjY21p+cFPAhI3MFdE/viHfifFOLlJmInmUnEn1WLidt8wlssk0zJMblTEG7rZq1BZw1GzOhHWfgMQIOtGzA5r7kumcAGSHYOHXpiSDoRwBqTAcvdacdOwlRZuc2s7dWKK7qSuF8v4eIcakqUa+V6q1BDhBlXbxq1wesunnQx3tLMYQagcHUxSpBEnqCxAztmTzzAZ/Vlk7kLQW/1JbVmcxHD2Ri6T2soAR5tiBVDjFShnR9TWeXPeaHmvAyQxWn05sSgtXTaJErSG/hRS1xpT/41IKoxumsqtMTWJPSa72xsW8PV8tKUnj2mK9jEe9U/l8zYDUhY8vtIUXU244mqz+9WXFfosYN/hKro5/aNQ2u6sx0bq6afldoqArbc+Dtm/EUeREaGET3n/rbc+4gP+iPgNo04Ue6Gbq9gAAAABJRU5ErkJggg=="></a>
<a href='https://docs.spring.io/spring-boot/docs/3.0.0-SNAPSHOT/reference/html'><img alt="Spring Boot 3" src="https://img.shields.io/badge/Spring%20Boot%203-%23000000.svg?logo=springboot"></a>
<a href='https://staging-cn.vuejs.org'><img alt="Vue 3" src="https://img.shields.io/badge/Vue%203%20-%232b3847.svg?logo=vue.js"></a><br/>
<a href='https://github.com/201206030/novel-cloud'><img alt="Github stars" src="https://img.shields.io/github/stars/201206030/novel-cloud?logo=github"></a>
<a href='https://github.com/201206030/novel-cloud'><img alt="Github forks" src="https://img.shields.io/github/forks/201206030/novel-cloud?logo=github"></a>
<a href='https://gitee.com/novel_dev_team/novel-cloud'><img alt="Gitee stars" src="https://gitee.com/novel_dev_team/novel-cloud/badge/star.svg?theme=gitee"></a>
<a href='https://gitee.com/novel_dev_team/novel-cloud'><img alt="Gitee forks" src="https://gitee.com/novel_dev_team/novel-cloud/badge/fork.svg?theme=gitee"></a>
<a href="https://github.com/201206030/novel-cloud"><img src="https://visitor-badge.glitch.me/badge?page_id=201206030.novel-cloud" alt="visitors"></a>
</p>
## 项目背景
小说网站业务难度适中,没有商城系统那种复杂的业务。但是作为互联网项目,一样需要面对大规模用户和海量数据的处理,所以高并发、高可用、高性能、高容错、可扩展性、可维护性也是小说网站设计需要考虑的问题,商城系统中所用到的技术同样适用于小说网站。
综上所述,使用微服务架构来构建一个小说门户平台是非常有必要的,利用微服务构建的小说门户平台来学习现下流行技术相较于业务比较复杂的商城系统来说也是比较容易的,非常适合于没有实际微服务项目经验的同学用来学习和入门微服务技术栈。
## 项目简介
novel 是一套基于时下最新 Java 技术栈 `Spring Boot 3` + `Vue 3` 开发的前后端分离学习型小说项目,配备 [保姆级教程](https://docs.xxyopen.com/course/novel) 手把手教你从零开始开发上线一套生产级别的 Java
系统,由小说门户系统、作家后台管理系统、平台后台管理系统等多个子系统构成。包括小说推荐、作品检索、小说排行榜、小说阅读、小说评论、会员中心、作家专区、充值订阅、新闻发布等功能。
novel-cloud 是 novel 项目的微服务版本,基于 `Spring Cloud 2022` & `Spring Cloud Alibaba 2022` 构建,数据结构、后端接口和 novel 项目保持完全一致,Vue 3 开发的前端能无缝对接这两个项目。
## 项目地址
- 单体架构后端项目:[GitHub](https://github.com/201206030/novel) | [码云](https://gitee.com/novel_dev_team/novel) | [文档](https://docs.xxyopen.com/course/novel)
- 微服务架构后端项目:[GitHub](https://github.com/201206030/novel-cloud) | [码云](https://gitee.com/novel_dev_team/novel-cloud) | [文档](https://docs.xxyopen.com/course/novelcloud)
- 前端项目:[GitHub](https://github.com/201206030/novel-front-web) | [码云](https://gitee.com/novel_dev_team/novel-front-web)
- 线上应用版:[GitHub](https://github.com/201206030/novel-plus) | [码云](https://gitee.com/novel_dev_team/novel-plus)
## 开发环境
- MySQL 8.0
- Redis 7.0
- Elasticsearch 8.6.2
- RabbitMQ 3.x
- XXL-JOB 2.3.1
- Nacos 2.2.1
- JDK 17
- Maven 3.8
- IntelliJ IDEA 2021.3(可选)
- Node 16.14
## 后端技术选型
| 技术 | 版本 | 说明 | 官网 | 学习 |
|----------------------|:--------------:|-----------------------------------| -------------------------------------- |:-----------------------------------------------------------------------------------------------------------------------------:|
| Spring Cloud | 2022.0.1 | 微服务开发的一站式解决方案 | [进入](https://spring.io/projects/spring-cloud) | [进入](https://docs.spring.io/spring-cloud/docs/current/reference/html/) |
| Spring Cloud Alibaba | 2022.0.0.0-RC1 | 阿里巴巴贡献的 Spring Cloud 微服务开发一站式解决方案 | [进入](https://github.com/alibaba/spring-cloud-alibaba) | [进入](https://spring-cloud-alibaba-group.github.io/github-pages/2021/zh-cn/2021.0.5.0/index.html) |
| Nacos | 2.2.1 | 服务发现和配置管理 | [进入](https://nacos.io) | [进入](https://nacos.io/zh-cn/docs/what-is-nacos.html) |
| Spring Boot Admin | 3.0.2 | 微服务管理和监控 | [进入](https://github.com/codecentric/spring-boot-admin) | [进入](https://codecentric.github.io/spring-boot-admin/3.0.0-M1) |
| Spring Boot | 3.0.5 | 容器 + MVC 框架 | [进入](https://spring.io/projects/spring-boot) | [进入](https://docs.spring.io/spring-boot/docs/3.0.0/reference/html) |
| MyBatis | 3.5.9 | ORM 框架 | [进入](http://www.mybatis.org) | [进入](https://mybatis.org/mybatis-3/zh/index.html) |
| MyBatis-Plus | 3.5.3 | MyBatis 增强工具 | [进入](https://baomidou.com/) | [进入](https://baomidou.com/pages/24112f/) |
| JJWT | 0.11.5 | JWT 登录支持 | [进入](https://github.com/jwtk/jjwt) | - |
| Lombok | 1.18.24 | 简化对象封装工具 | [进入](https://github.com/projectlombok/lombok) | [进入](https://projectlombok.org/features/all) |
| Caffeine | 3.1.0 | 本地缓存支持 | [进入](https://github.com/ben-manes/caffeine) | [进入](https://github.com/ben-manes/caffeine/wiki/Home-zh-CN) |
| Redis | 7.0 | 分布式缓存支持 | [进入](https://redis.io) | [进入](https://redis.io/docs) |
| Redisson | 3.17.4 | 分布式锁实现 | [进入](https://github.com/redisson/redisson) | [进入](https://github.com/redisson/redisson/wiki/%E7%9B%AE%E5%BD%95) |
| RabbitMQ | 3.x | 开源消息中间件 | [进入](https://www.rabbitmq.com) | [进入](https://www.rabbitmq.com/tutorials/tutorial-one-java.html) |
| MySQL | 8.0 | 数据库服务 | [进入](https://www.mysql.com) | [进入](https://docs.oracle.com/en-us/iaas/mysql-database/doc/getting-started.html) |
| ShardingSphere-JDBC | 5.1.1 | 数据库分库分表支持 | [进入](https://shardingsphere.apache.org) | [进入](https://shardingsphere.apache.org/document/5.1.1/cn/overview) |
| Elasticsearch | 8.6.2 | 搜索引擎服务 | [进入](https://www.elastic.co) | [进入](https://www.elastic.co/guide/en/elasticsearch/reference/current/index.html) |
| XXL-JOB | 2.3.1 | 分布式任务调度平台 | [进入](https://www.xuxueli.com/xxl-job) | [进入](https://www.xuxueli.com/xxl-job) |
| Springdoc-openapi | 2.0.0 | Swagger 3 接口文档自动生成 | [进入](https://github.com/springdoc/springdoc-openapi) | [进入](https://springdoc.org/) |
| Undertow | 2.2.17.Final | Java 开发的高性能 Web 服务器 | [进入](https://undertow.io) | [进入](https://undertow.io/documentation.html) |
**注:更多热门新技术待集成。**
## 前端技术选型
| 技术 | 版本 | 说明 | 官网 | 学习 |
| :----------------- | :-----: | -------------------------- |--------------------------------| :-------------------------------------------------: |
| Vue.js | 3.2.13 | 渐进式 JavaScript 框架 | [进入](https://vuejs.org) | [进入](https://staging-cn.vuejs.org/guide/introduction.html) |
| Vue Router | 4.0.15 | Vue.js 的官方路由 | [进入](https://router.vuejs.org) | [进入](https://router.vuejs.org/zh/guide/) |
| axios | 0.27.2 | 基于 promise 的网络请求库 | [进入](https://axios-http.com) | [进入](https://axios-http.com/zh/docs/intro) |
| element-plus | 2.2.0 | 基于 Vue 3,面向设计师和开发者的组件库 | [进入](https://element-plus.org) | [进入](https://element-plus.org/zh-CN/guide/design.html) |
## 软件架构
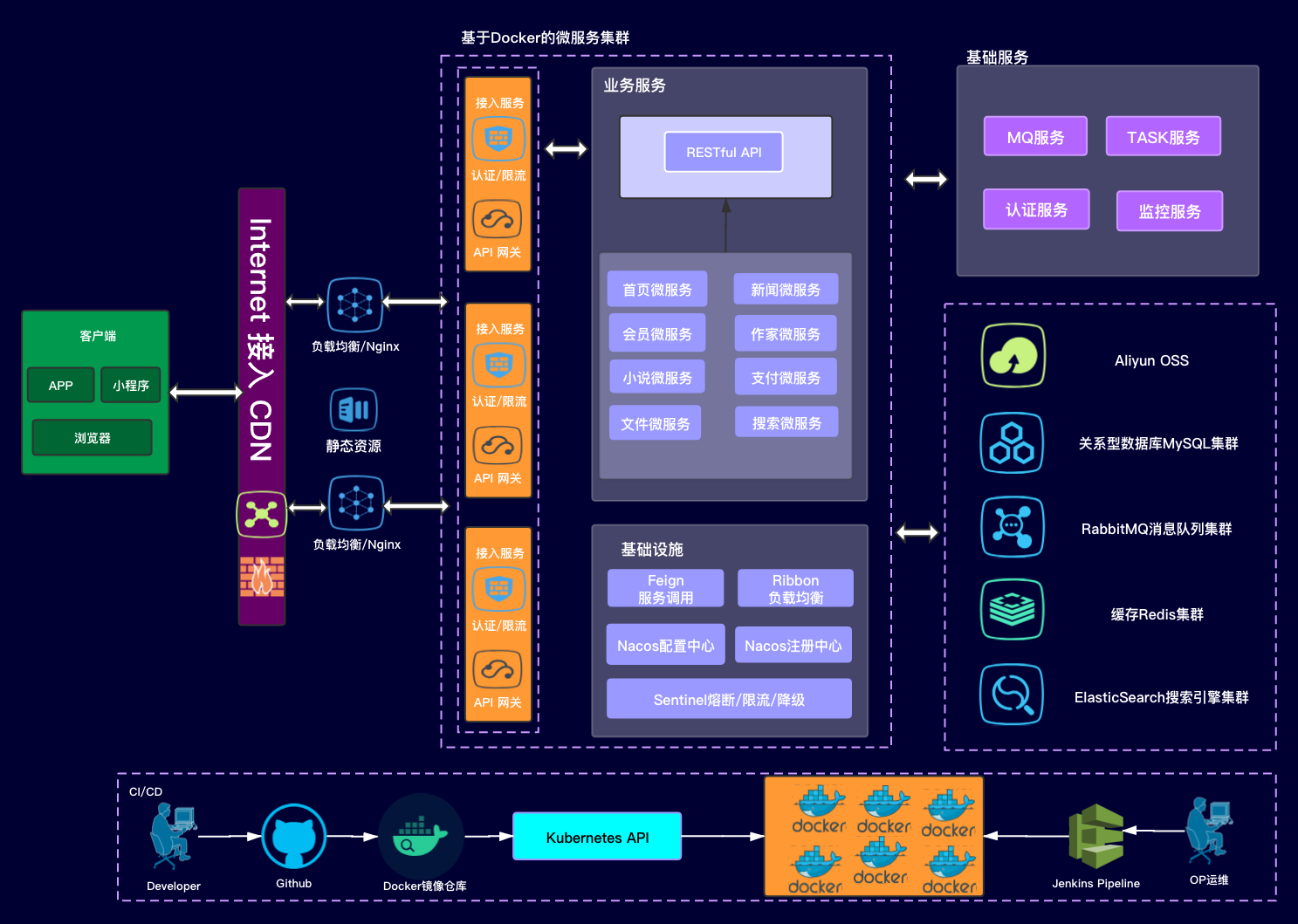
👉 [克隆架构图](https://www.processon.com/view/5fd028fbe0b34d425254e710)
## 项目结构
```
novel-cloud
├── novel-core -- 项目核心模块,供其它各个业务微服务依赖
├── novel-gateway -- 基于 Spring Cloud Gateway 构建的微服务网关
├── novel-monitor -- 基于 Spring Boot Admin 构建的微服务监控中心
├── novel-search -- 基于 Elasticsearch 构建的搜索服务
├── novel-home -- 首页微服务
├── novel-news -- 新闻微服务
├── novel-book -- 小说微服务
├── novel-user -- 会员微服务
├── novel-author -- 作家微服务
└── novel-resource -- 资源微服务
```
## 项目演示
[https://www.bilibili.com/video/BV1Xa4y1T7CF](https://www.bilibili.com/video/BV1Xa4y1T7CF/)
## 捐赠支持
开源项目不易,若此项目能得到你的青睐,可以捐赠支持作者持续开发与维护。
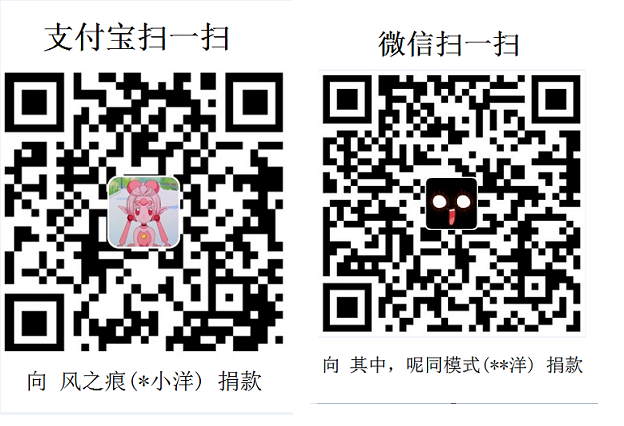
| 0 |
aptana/studio3 | This repository contains the code for core components of Aptana Studio 3. | null | [](https://jenkins.appcelerator.org/job/aptana-studio/job/studio3/job/development/)
Aptana Studio 3 Core
========================
Aptana Studio 3 Core holds the core set of plugins used to build the Aptana Studio 3 IDE/RCP. Currently it relies on consuming a pre-built [FTP/FTPS/SFTP library](https://github.com/aptana/libraries_com) as a dependency for building. This holds a commercial library, so the sources do not contain the JAR due to licensing restrictions.
This set of plugins deals with providing support for web development: HTML, CSS, XML, and JavaScript. It also includes our own Git plugin support. Features that deal with adding support for [PHP](https://github.com/aptana/studio3-php), [Python](https://github.com/aptana/PyDev) and [Ruby](https://github.com/aptana/studio3-ruby) are broken out to other repositories. The Portion dealing with bundling all of that together to form the IDE is in the [studio3-rcp](https://github.com/aptana/studio3-rcp) repository.
How to Download Aptana
----------
1. Visit https://github.com/aptana/studio3/releases
2. Download the latest version for your operating system. Windows (.exe) and Mac (.dmg) have installers. Linux is a .zip file that you'll expand in your chosen location.
How to Contribute
----------
1. Fork this repo.
2. Ensure you have [Maven 3.x+ installed](http://www.vogella.com/tutorials/ApacheMaven/article.html#maven_installation)
3. Make local changes and test with maven/tycho:
```mvn clean verify```
4. Submit a PR
Setting up an Eclipse Development Environment
----------------------------------------------
2. [Download Eclipse 4.13 for RCP Developers](https://www.eclipse.org/downloads/packages/release/2019-09/r#node-55605) and install it
3. Install npm packages for our dependency installer script: ```npm ci```
4. Install the dependencies from the target platform into eclipse ```npm run eclipse```
5. Then import the features, plugins, and test plugins into your workspace. _File > Import > General > Existing Projects Into Workspace_. Select the directory this repo was cloned into.
6. Make your changes on your fork, then submit a Pull Request.
License
-------
This program Copyright (c) 2005-2019 by Appcelerator, Inc. This program is distributed under the GNU General Public license. This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License, Version 3, as published by the Free Software Foundation.
Any modifications must keep this entire license intact.
-----------------------------------------------------------------------
GNU General Public License
Version 3, 29 June 2007
Copyright (C) 2007 Free Software Foundation, Inc. http://fsf.org/
Everyone is permitted to copy and distribute verbatim copies of this license document, but changing it is not allowed.
Preamble
The GNU General Public License is a free, copyleft license for software and other kinds of works.
The licenses for most software and other practical works are designed to take away your freedom to share and change the works. By contrast, the GNU General Public License is intended to guarantee your freedom to share and change all versions of a program--to make sure it remains free software for all its users. We, the Free Software Foundation, use the GNU General Public License for most of our software; it applies also to any other work released this way by its authors. You can apply it to your programs, too.
When we speak of free software, we are referring to freedom, not price. Our General Public Licenses are designed to make sure that you have the freedom to distribute copies of free software (and charge for them if you wish), that you receive source code or can get it if you want it, that you can change the software or use pieces of it in new free programs, and that you know you can do these things.
To protect your rights, we need to prevent others from denying you these rights or asking you to surrender the rights. Therefore, you have certain responsibilities if you distribute copies of the software, or if you modify it: responsibilities to respect the freedom of others.
For example, if you distribute copies of such a program, whether gratis or for a fee, you must pass on to the recipients the same freedoms that you received. You must make sure that they, too, receive or can get the source code. And you must show them these terms so they know their rights.
Developers that use the GNU GPL protect your rights with two steps: (1) assert copyright on the software, and (2) offer you this License giving you legal permission to copy, distribute and/or modify it.
For the developers' and authors' protection, the GPL clearly explains that there is no warranty for this free software. For both users' and authors' sake, the GPL requires that modified versions be marked as changed, so that their problems will not be attributed erroneously to authors of previous versions.
Some devices are designed to deny users access to install or run modified versions of the software inside them, although the manufacturer can do so. This is fundamentally incompatible with the aim of protecting users' freedom to change the software. The systematic pattern of such abuse occurs in the area of products for individuals to use, which is precisely where it is most unacceptable. Therefore, we have designed this version of the GPL to prohibit the practice for those products. If such problems arise substantially in other domains, we stand ready to extend this provision to those domains in future versions of the GPL, as needed to protect the freedom of users.
Finally, every program is threatened constantly by software patents. States should not allow patents to restrict development and use of software on general-purpose computers, but in those that do, we wish to avoid the special danger that patents applied to a free program could make it effectively proprietary. To prevent this, the GPL assures that patents cannot be used to render the program non-free.
The precise terms and conditions for copying, distribution and modification follow.
TERMS AND CONDITIONS
0. Definitions.
"This License" refers to version 3 of the GNU General Public License.
"Copyright" also means copyright-like laws that apply to other kinds of works, such as semiconductor masks.
"The Program" refers to any copyrightable work licensed under this License. Each licensee is addressed as "you". "Licensees" and "recipients" may be individuals or organizations.
To "modify" a work means to copy from or adapt all or part of the work in a fashion requiring copyright permission, other than the making of an exact copy. The resulting work is called a "modified version" of the earlier work or a work "based on" the earlier work.
A "covered work" means either the unmodified Program or a work based on the Program.
To "propagate" a work means to do anything with it that, without permission, would make you directly or secondarily liable for infringement under applicable copyright law, except executing it on a computer or modifying a private copy. Propagation includes copying, distribution (with or without modification), making available to the public, and in some countries other activities as well.
To "convey" a work means any kind of propagation that enables other parties to make or receive copies. Mere interaction with a user through a computer network, with no transfer of a copy, is not conveying.
An interactive user interface displays "Appropriate Legal Notices" to the extent that it includes a convenient and prominently visible feature that (1) displays an appropriate copyright notice, and (2) tells the user that there is no warranty for the work (except to the extent that warranties are provided), that licensees may convey the work under this License, and how to view a copy of this License. If the interface presents a list of user commands or options, such as a menu, a prominent item in the list meets this criterion.
1. Source Code.
The "source code" for a work means the preferred form of the work for making modifications to it. "Object code" means any non-source form of a work.
A "Standard Interface" means an interface that either is an official standard defined by a recognized standards body, or, in the case of interfaces specified for a particular programming language, one that is widely used among developers working in that language.
The "System Libraries" of an executable work include anything, other than the work as a whole, that (a) is included in the normal form of packaging a Major Component, but which is not part of that Major Component, and (b) serves only to enable use of the work with that Major Component, or to implement a Standard Interface for which an implementation is available to the public in source code form. A "Major Component", in this context, means a major essential component (kernel, window system, and so on) of the specific operating system (if any) on which the executable work runs, or a compiler used to produce the work, or an object code interpreter used to run it.
The "Corresponding Source" for a work in object code form means all the source code needed to generate, install, and (for an executable work) run the object code and to modify the work, including scripts to control those activities. However, it does not include the work's System Libraries, or general-purpose tools or generally available free programs which are used unmodified in performing those activities but which are not part of the work. For example, Corresponding Source includes interface definition files associated with source files for the work, and the source code for shared libraries and dynamically linked subprograms that the work is specifically designed to require, such as by intimate data communication or control flow between those subprograms and other parts of the work.
The Corresponding Source need not include anything that users can regenerate automatically from other parts of the Corresponding Source.
The Corresponding Source for a work in source code form is that same work.
2. Basic Permissions.
All rights granted under this License are granted for the term of copyright on the Program, and are irrevocable provided the stated conditions are met. This License explicitly affirms your unlimited permission to run the unmodified Program. The output from running a covered work is covered by this License only if the output, given its content, constitutes a covered work. This License acknowledges your rights of fair use or other equivalent, as provided by copyright law.
You may make, run and propagate covered works that you do not convey, without conditions so long as your license otherwise remains in force. You may convey covered works to others for the sole purpose of having them make modifications exclusively for you, or provide you with facilities for running those works, provided that you comply with the terms of this License in conveying all material for which you do not control copyright. Those thus making or running the covered works for you must do so exclusively on your behalf, under your direction and control, on terms that prohibit them from making any copies of your copyrighted material outside their relationship with you.
Conveying under any other circumstances is permitted solely under the conditions stated below. Sublicensing is not allowed; section 10 makes it unnecessary.
3. Protecting Users' Legal Rights From Anti-Circumvention Law.
No covered work shall be deemed part of an effective technological measure under any applicable law fulfilling obligations under article 11 of the WIPO copyright treaty adopted on 20 December 1996, or similar laws prohibiting or restricting circumvention of such measures.
When you convey a covered work, you waive any legal power to forbid circumvention of technological measures to the extent such circumvention is effected by exercising rights under this License with respect to the covered work, and you disclaim any intention to limit operation or modification of the work as a means of enforcing, against the work's users, your or third parties' legal rights to forbid circumvention of technological measures.
4. Conveying Verbatim Copies.
You may convey verbatim copies of the Program's source code as you receive it, in any medium, provided that you conspicuously and appropriately publish on each copy an appropriate copyright notice; keep intact all notices stating that this License and any non-permissive terms added in accord with section 7 apply to the code; keep intact all notices of the absence of any warranty; and give all recipients a copy of this License along with the Program.
You may charge any price or no price for each copy that you convey, and you may offer support or warranty protection for a fee.
5. Conveying Modified Source Versions.
You may convey a work based on the Program, or the modifications to produce it from the Program, in the form of source code under the terms of section 4, provided that you also meet all of these conditions:
* a) The work must carry prominent notices stating that you modified it, and giving a relevant date.
* b) The work must carry prominent notices stating that it is released under this License and any conditions added under section 7. This requirement modifies the requirement in section 4 to "keep intact all notices".
* c) You must license the entire work, as a whole, under this License to anyone who comes into possession of a copy. This License will therefore apply, along with any applicable section 7 additional terms, to the whole of the work, and all its parts, regardless of how they are packaged. This License gives no permission to license the work in any other way, but it does not invalidate such permission if you have separately received it.
* d) If the work has interactive user interfaces, each must display Appropriate Legal Notices; however, if the Program has interactive interfaces that do not display Appropriate Legal Notices, your work need not make them do so.
A compilation of a covered work with other separate and independent works, which are not by their nature extensions of the covered work, and which are not combined with it such as to form a larger program, in or on a volume of a storage or distribution medium, is called an "aggregate" if the compilation and its resulting copyright are not used to limit the access or legal rights of the compilation's users beyond what the individual works permit. Inclusion of a covered work in an aggregate does not cause this License to apply to the other parts of the aggregate.
6. Conveying Non-Source Forms.
You may convey a covered work in object code form under the terms of sections 4 and 5, provided that you also convey the machine-readable Corresponding Source under the terms of this License, in one of these ways:
* a) Convey the object code in, or embodied in, a physical product (including a physical distribution medium), accompanied by the Corresponding Source fixed on a durable physical medium customarily used for software interchange.
* b) Convey the object code in, or embodied in, a physical product (including a physical distribution medium), accompanied by a written offer, valid for at least three years and valid for as long as you offer spare parts or customer support for that product model, to give anyone who possesses the object code either (1) a copy of the Corresponding Source for all the software in the product that is covered by this License, on a durable physical medium customarily used for software interchange, for a price no more than your reasonable cost of physically performing this conveying of source, or (2) access to copy the Corresponding Source from a network server at no charge.
* c) Convey individual copies of the object code with a copy of the written offer to provide the Corresponding Source. This alternative is allowed only occasionally and noncommercially, and only if you received the object code with such an offer, in accord with subsection 6b.
* d) Convey the object code by offering access from a designated place (gratis or for a charge), and offer equivalent access to the Corresponding Source in the same way through the same place at no further charge. You need not require recipients to copy the Corresponding Source along with the object code. If the place to copy the object code is a network server, the Corresponding Source may be on a different server (operated by you or a third party) that supports equivalent copying facilities, provided you maintain clear directions next to the object code saying where to find the Corresponding Source. Regardless of what server hosts the Corresponding Source, you remain obligated to ensure that it is available for as long as needed to satisfy these requirements.
* e) Convey the object code using peer-to-peer transmission, provided you inform other peers where the object code and Corresponding Source of the work are being offered to the general public at no charge under subsection 6d.
A separable portion of the object code, whose source code is excluded from the Corresponding Source as a System Library, need not be included in conveying the object code work.
A "User Product" is either (1) a "consumer product", which means any tangible personal property which is normally used for personal, family, or household purposes, or (2) anything designed or sold for incorporation into a dwelling. In determining whether a product is a consumer product, doubtful cases shall be resolved in favor of coverage. For a particular product received by a particular user, "normally used" refers to a typical or common use of that class of product, regardless of the status of the particular user or of the way in which the particular user actually uses, or expects or is expected to use, the product. A product is a consumer product regardless of whether the product has substantial commercial, industrial or non-consumer uses, unless such uses represent the only significant mode of use of the product.
"Installation Information" for a User Product means any methods, procedures, authorization keys, or other information required to install and execute modified versions of a covered work in that User Product from a modified version of its Corresponding Source. The information must suffice to ensure that the continued functioning of the modified object code is in no case prevented or interfered with solely because modification has been made.
If you convey an object code work under this section in, or with, or specifically for use in, a User Product, and the conveying occurs as part of a transaction in which the right of possession and use of the User Product is transferred to the recipient in perpetuity or for a fixed term (regardless of how the transaction is characterized), the Corresponding Source conveyed under this section must be accompanied by the Installation Information. But this requirement does not apply if neither you nor any third party retains the ability to install modified object code on the User Product (for example, the work has been installed in ROM).
The requirement to provide Installation Information does not include a requirement to continue to provide support service, warranty, or updates for a work that has been modified or installed by the recipient, or for the User Product in which it has been modified or installed. Access to a network may be denied when the modification itself materially and adversely affects the operation of the network or violates the rules and protocols for communication across the network.
Corresponding Source conveyed, and Installation Information provided, in accord with this section must be in a format that is publicly documented (and with an implementation available to the public in source code form), and must require no special password or key for unpacking, reading or copying.
7. Additional Terms.
"Additional permissions" are terms that supplement the terms of this License by making exceptions from one or more of its conditions. Additional permissions that are applicable to the entire Program shall be treated as though they were included in this License, to the extent that they are valid under applicable law. If additional permissions apply only to part of the Program, that part may be used separately under those permissions, but the entire Program remains governed by this License without regard to the additional permissions.
When you convey a copy of a covered work, you may at your option remove any additional permissions from that copy, or from any part of it. (Additional permissions may be written to require their own removal in certain cases when you modify the work.) You may place additional permissions on material, added by you to a covered work, for which you have or can give appropriate copyright permission.
Notwithstanding any other provision of this License, for material you add to a covered work, you may (if authorized by the copyright holders of that material) supplement the terms of this License with terms:
* a) Disclaiming warranty or limiting liability differently from the terms of sections 15 and 16 of this License; or
* b) Requiring preservation of specified reasonable legal notices or author attributions in that material or in the Appropriate Legal Notices displayed by works containing it; or
* c) Prohibiting misrepresentation of the origin of that material, or requiring that modified versions of such material be marked in reasonable ways as different from the original version; or
* d) Limiting the use for publicity purposes of names of licensors or authors of the material; or
* e) Declining to grant rights under trademark law for use of some trade names, trademarks, or service marks; or
* f) Requiring indemnification of licensors and authors of that material by anyone who conveys the material (or modified versions of it) with contractual assumptions of liability to the recipient, for any liability that these contractual assumptions directly impose on those licensors and authors.
All other non-permissive additional terms are considered "further restrictions" within the meaning of section 10. If the Program as you received it, or any part of it, contains a notice stating that it is governed by this License along with a term that is a further restriction, you may remove that term. If a license document contains a further restriction but permits relicensing or conveying under this License, you may add to a covered work material governed by the terms of that license document, provided that the further restriction does not survive such relicensing or conveying.
If you add terms to a covered work in accord with this section, you must place, in the relevant source files, a statement of the additional terms that apply to those files, or a notice indicating where to find the applicable terms.
Additional terms, permissive or non-permissive, may be stated in the form of a separately written license, or stated as exceptions; the above requirements apply either way.
8. Termination.
You may not propagate or modify a covered work except as expressly provided under this License. Any attempt otherwise to propagate or modify it is void, and will automatically terminate your rights under this License (including any patent licenses granted under the third paragraph of section 11).
However, if you cease all violation of this License, then your license from a particular copyright holder is reinstated (a) provisionally, unless and until the copyright holder explicitly and finally terminates your license, and (b) permanently, if the copyright holder fails to notify you of the violation by some reasonable means prior to 60 days after the cessation.
Moreover, your license from a particular copyright holder is reinstated permanently if the copyright holder notifies you of the violation by some reasonable means, this is the first time you have received notice of violation of this License (for any work) from that copyright holder, and you cure the violation prior to 30 days after your receipt of the notice.
Termination of your rights under this section does not terminate the licenses of parties who have received copies or rights from you under this License. If your rights have been terminated and not permanently reinstated, you do not qualify to receive new licenses for the same material under section 10.
9. Acceptance Not Required for Having Copies.
You are not required to accept this License in order to receive or run a copy of the Program. Ancillary propagation of a covered work occurring solely as a consequence of using peer-to-peer transmission to receive a copy likewise does not require acceptance. However, nothing other than this License grants you permission to propagate or modify any covered work. These actions infringe copyright if you do not accept this License. Therefore, by modifying or propagating a covered work, you indicate your acceptance of this License to do so.
10. Automatic Licensing of Downstream Recipients.
Each time you convey a covered work, the recipient automatically receives a license from the original licensors, to run, modify and propagate that work, subject to this License. You are not responsible for enforcing compliance by third parties with this License.
An "entity transaction" is a transaction transferring control of an organization, or substantially all assets of one, or subdividing an organization, or merging organizations. If propagation of a covered work results from an entity transaction, each party to that transaction who receives a copy of the work also receives whatever licenses to the work the party's predecessor in interest had or could give under the previous paragraph, plus a right to possession of the Corresponding Source of the work from the predecessor in interest, if the predecessor has it or can get it with reasonable efforts.
You may not impose any further restrictions on the exercise of the rights granted or affirmed under this License. For example, you may not impose a license fee, royalty, or other charge for exercise of rights granted under this License, and you may not initiate litigation (including a cross-claim or counterclaim in a lawsuit) alleging that any patent claim is infringed by making, using, selling, offering for sale, or importing the Program or any portion of it.
11. Patents.
A "contributor" is a copyright holder who authorizes use under this License of the Program or a work on which the Program is based. The work thus licensed is called the contributor's "contributor version".
A contributor's "essential patent claims" are all patent claims owned or controlled by the contributor, whether already acquired or hereafter acquired, that would be infringed by some manner, permitted by this License, of making, using, or selling its contributor version, but do not include claims that would be infringed only as a consequence of further modification of the contributor version. For purposes of this definition, "control" includes the right to grant patent sublicenses in a manner consistent with the requirements of this License.
Each contributor grants you a non-exclusive, worldwide, royalty-free patent license under the contributor's essential patent claims, to make, use, sell, offer for sale, import and otherwise run, modify and propagate the contents of its contributor version.
In the following three paragraphs, a "patent license" is any express agreement or commitment, however denominated, not to enforce a patent (such as an express permission to practice a patent or covenant not to sue for patent infringement). To "grant" such a patent license to a party means to make such an agreement or commitment not to enforce a patent against the party.
If you convey a covered work, knowingly relying on a patent license, and the Corresponding Source of the work is not available for anyone to copy, free of charge and under the terms of this License, through a publicly available network server or other readily accessible means, then you must either (1) cause the Corresponding Source to be so available, or (2) arrange to deprive yourself of the benefit of the patent license for this particular work, or (3) arrange, in a manner consistent with the requirements of this License, to extend the patent license to downstream recipients. "Knowingly relying" means you have actual knowledge that, but for the patent license, your conveying the covered work in a country, or your recipient's use of the covered work in a country, would infringe one or more identifiable patents in that country that you have reason to believe are valid.
If, pursuant to or in connection with a single transaction or arrangement, you convey, or propagate by procuring conveyance of, a covered work, and grant a patent license to some of the parties receiving the covered work authorizing them to use, propagate, modify or convey a specific copy of the covered work, then the patent license you grant is automatically extended to all recipients of the covered work and works based on it.
A patent license is "discriminatory" if it does not include within the scope of its coverage, prohibits the exercise of, or is conditioned on the non-exercise of one or more of the rights that are specifically granted under this License. You may not convey a covered work if you are a party to an arrangement with a third party that is in the business of distributing software, under which you make payment to the third party based on the extent of your activity of conveying the work, and under which the third party grants, to any of the parties who would receive the covered work from you, a discriminatory patent license (a) in connection with copies of the covered work conveyed by you (or copies made from those copies), or (b) primarily for and in connection with specific products or compilations that contain the covered work, unless you entered into that arrangement, or that patent license was granted, prior to 28 March 2007.
Nothing in this License shall be construed as excluding or limiting any implied license or other defenses to infringement that may otherwise be available to you under applicable patent law.
12. No Surrender of Others' Freedom.
If conditions are imposed on you (whether by court order, agreement or otherwise) that contradict the conditions of this License, they do not excuse you from the conditions of this License. If you cannot convey a covered work so as to satisfy simultaneously your obligations under this License and any other pertinent obligations, then as a consequence you may not convey it at all. For example, if you agree to terms that obligate you to collect a royalty for further conveying from those to whom you convey the Program, the only way you could satisfy both those terms and this License would be to refrain entirely from conveying the Program.
13. Use with the GNU Affero General Public License.
Notwithstanding any other provision of this License, you have permission to link or combine any covered work with a work licensed under version 3 of the GNU Affero General Public License into a single combined work, and to convey the resulting work. The terms of this License will continue to apply to the part which is the covered work, but the special requirements of the GNU Affero General Public License, section 13, concerning interaction through a network will apply to the combination as such.
14. Revised Versions of this License.
The Free Software Foundation may publish revised and/or new versions of the GNU General Public License from time to time. Such new versions will be similar in spirit to the present version, but may differ in detail to address new problems or concerns.
Each version is given a distinguishing version number. If the Program specifies that a certain numbered version of the GNU General Public License "or any later version" applies to it, you have the option of following the terms and conditions either of that numbered version or of any later version published by the Free Software Foundation. If the Program does not specify a version number of the GNU General Public License, you may choose any version ever published by the Free Software Foundation.
If the Program specifies that a proxy can decide which future versions of the GNU General Public License can be used, that proxy's public statement of acceptance of a version permanently authorizes you to choose that version for the Program.
Later license versions may give you additional or different permissions. However, no additional obligations are imposed on any author or copyright holder as a result of your choosing to follow a later version.
15. Disclaimer of Warranty.
THERE IS NO WARRANTY FOR THE PROGRAM, TO THE EXTENT PERMITTED BY APPLICABLE LAW. EXCEPT WHEN OTHERWISE STATED IN WRITING THE COPYRIGHT HOLDERS AND/OR OTHER PARTIES PROVIDE THE PROGRAM "AS IS" WITHOUT WARRANTY OF ANY KIND, EITHER EXPRESSED OR IMPLIED, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE. THE ENTIRE RISK AS TO THE QUALITY AND PERFORMANCE OF THE PROGRAM IS WITH YOU. SHOULD THE PROGRAM PROVE DEFECTIVE, YOU ASSUME THE COST OF ALL NECESSARY SERVICING, REPAIR OR CORRECTION.
16. Limitation of Liability.
IN NO EVENT UNLESS REQUIRED BY APPLICABLE LAW OR AGREED TO IN WRITING WILL ANY COPYRIGHT HOLDER, OR ANY OTHER PARTY WHO MODIFIES AND/OR CONVEYS THE PROGRAM AS PERMITTED ABOVE, BE LIABLE TO YOU FOR DAMAGES, INCLUDING ANY GENERAL, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES ARISING OUT OF THE USE OR INABILITY TO USE THE PROGRAM (INCLUDING BUT NOT LIMITED TO LOSS OF DATA OR DATA BEING RENDERED INACCURATE OR LOSSES SUSTAINED BY YOU OR THIRD PARTIES OR A FAILURE OF THE PROGRAM TO OPERATE WITH ANY OTHER PROGRAMS), EVEN IF SUCH HOLDER OR OTHER PARTY HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES.
17. Interpretation of Sections 15 and 16.
If the disclaimer of warranty and limitation of liability provided above cannot be given local legal effect according to their terms, reviewing courts shall apply local law that most closely approximates an absolute waiver of all civil liability in connection with the Program, unless a warranty or assumption of liability accompanies a copy of the Program in return for a fee.
END OF TERMS AND CONDITIONS
-----------------------------------------------------------------------
Appcelerator GPL Exception
Section 7 Exception
As a special exception to the terms and conditions of the GNU General Public License Version 3 (the "GPL"): You are free to convey a modified version that is formed entirely from this file (for purposes of this exception, the "Program" under the GPL) and the works identified at http://www.aptana.com/legal/gpl (each an "Excepted Work"), which are conveyed to you by Appcelerator, Inc. and licensed under one or more of the licenses identified in the Excepted License List below (each an "Excepted License"), as long as:
1. you obey the GPL in all respects for the Program and the modified version, except for Excepted Works which are identifiable sections of the modified version, which are not derived from the Program, and which can reasonably be considered independent and separate works in themselves,
2. all Excepted Works which are identifiable sections of the modified version, which are not derived from the Program, and which can reasonably be considered independent and separate works in themselves,
1. are distributed subject to the Excepted License under which they were originally licensed, and
2. are not themselves modified from the form in which they are conveyed to you by Aptana, and
3. the object code or executable form of those sections are accompanied by the complete corresponding machine-readable source code for those sections, on the same medium as the corresponding object code or executable forms of those sections, and are licensed under the applicable Excepted License as the corresponding object code or executable forms of those sections, and
3. any works which are aggregated with the Program, or with a modified version on a volume of a storage or distribution medium in accordance with the GPL, are aggregates (as defined in Section 5 of the GPL) which can reasonably be considered independent and separate works in themselves and which are not modified versions of either the Program, a modified version, or an Excepted Work.
If the above conditions are not met, then the Program may only be copied, modified, distributed or used under the terms and conditions of the GPL or another valid licensing option from Appcelerator, Inc. Terms used but not defined in the foregoing paragraph have the meanings given in the GPL.
-----------------------------------------------------------------------
Excepted License List
* Apache Software License: version 1.0, 1.1, 2.0
* Eclipse Public License: version 1.0
* GNU General Public License: version 2.0
* GNU Lesser General Public License: version 2.0
* License of Jaxer
* License of HTML jTidy
* Mozilla Public License: version 1.1
* W3C License
* BSD License
* MIT License
* Aptana Commercial Licenses
This list may be modified by Appcelerator from time to time. See Appcelerator's website for the latest version.
-----------------------------------------------------------------------
Attribution Requirement
This license does not grant any license or rights to use the trademarks "Aptana," any "Aptana" logos, or any other trademarks of Appcelerator, Inc. You are not authorized to use the name Aptana or the names of any author or contributor for publicity purposes, without written authorization.
However, in addition to the other notice obligations of this License, all copies of any covered work conveyed by you must include on each user interface screen and in the Appropriate Legal Notices the following text: "Powered by Aptana". On user interface screens, this text must be visibly and clearly displayed in the title bar, status bar, or otherwise directly in the view that is in focus.
| 0 |
spring-projects/spring-retry | null | null | [](https://ci.spring.io/teams/spring-retry/pipelines/spring-retry-2.0.x) [](https://www.javadoc.io/doc/org.springframework.retry/spring-retry)
This project provides declarative retry support for Spring
applications. It is used in Spring Batch, Spring Integration, and
others.
Imperative retry is also supported for explicit usage.
## Quick Start
This section provides a quick introduction to getting started with Spring Retry.
It includes a declarative example and an imperative example.
### Declarative Example
The following example shows how to use Spring Retry in its declarative style:
```java
@Configuration
@EnableRetry
public class Application {
}
@Service
class Service {
@Retryable(retryFor = RemoteAccessException.class)
public void service() {
// ... do something
}
@Recover
public void recover(RemoteAccessException e) {
// ... panic
}
}
```
This example calls the `service` method and, if it fails with a `RemoteAccessException`, retries
(by default, up to three times), and then tries the `recover` method if unsuccessful.
There are various options in the `@Retryable` annotation attributes for including and
excluding exception types, limiting the number of retries, and setting the policy for backoff.
The declarative approach to applying retry handling by using the `@Retryable` annotation shown earlier has an additional
runtime dependency on AOP classes. For details on how to resolve this dependency in your project, see the
['Java Configuration for Retry Proxies'](#javaConfigForRetryProxies) section.
### Imperative Example
The following example shows how to use Spring Retry in its imperative style (available since version 1.3):
```java
RetryTemplate template = RetryTemplate.builder()
.maxAttempts(3)
.fixedBackoff(1000)
.retryOn(RemoteAccessException.class)
.build();
template.execute(ctx -> {
// ... do something
});
```
For versions prior to 1.3,
see the examples in the [RetryTemplate](#using-retrytemplate) section.
## Building
Spring Retry requires Java 1.7 and Maven 3.0.5 (or greater).
To build, run the following Maven command:
```
$ mvn install
```
## Features and API
This section discusses the features of Spring Retry and shows how to use its API.
### Using `RetryTemplate`
To make processing more robust and less prone to failure, it sometimes helps to
automatically retry a failed operation, in case it might succeed on a subsequent attempt.
Errors that are susceptible to this kind of treatment are transient in nature. For
example, a remote call to a web service or an RMI service that fails because of a network
glitch or a `DeadLockLoserException` in a database update may resolve itself after a
short wait. To automate the retry of such operations, Spring Retry has the
`RetryOperations` strategy. The `RetryOperations` interface definition follows:
```java
public interface RetryOperations {
<T, E extends Throwable> T execute(RetryCallback<T, E> retryCallback) throws E;
<T, E extends Throwable> T execute(RetryCallback<T, E> retryCallback, RecoveryCallback<T> recoveryCallback) throws E;
<T, E extends Throwable> T execute(RetryCallback<T, E> retryCallback, RetryState retryState) throws E, ExhaustedRetryException;
<T, E extends Throwable> T execute(RetryCallback<T, E> retryCallback, RecoveryCallback<T> recoveryCallback, RetryState retryState) throws E;
}
```
The basic callback is a simple interface that lets you insert some business logic to be retried:
```java
public interface RetryCallback<T, E extends Throwable> {
T doWithRetry(RetryContext context) throws E;
}
```
The callback is tried, and, if it fails (by throwing an `Exception`), it is retried
until either it is successful or the implementation decides to abort. There are a number
of overloaded `execute` methods in the `RetryOperations` interface, to deal with various
use cases for recovery when all retry attempts are exhausted and to deal with retry state, which
lets clients and implementations store information between calls (more on this later).
The simplest general purpose implementation of `RetryOperations` is `RetryTemplate`.
The following example shows how to use it:
```java
RetryTemplate template = new RetryTemplate();
TimeoutRetryPolicy policy = new TimeoutRetryPolicy();
policy.setTimeout(30000L);
template.setRetryPolicy(policy);
MyObject result = template.execute(new RetryCallback<MyObject, Exception>() {
public MyObject doWithRetry(RetryContext context) {
// Do stuff that might fail, e.g. webservice operation
return result;
}
});
```
In the preceding example, we execute a web service call and return the result to the user.
If that call fails, it is retried until a timeout is reached.
Since version 1.3, fluent configuration of `RetryTemplate` is also available, as follows:
```java
RetryTemplate.builder()
.maxAttempts(10)
.exponentialBackoff(100, 2, 10000)
.retryOn(IOException.class)
.traversingCauses()
.build();
RetryTemplate.builder()
.fixedBackoff(10)
.withinMillis(3000)
.build();
RetryTemplate.builder()
.infiniteRetry()
.retryOn(IOException.class)
.uniformRandomBackoff(1000, 3000)
.build();
```
### Using `RetryContext`
The method parameter for the `RetryCallback` is a `RetryContext`.
Many callbacks ignore the context.
However, if necessary, you can use it as an attribute bag to store data for the duration of the iteration.
It also has some useful properties, such as `retryCount`.
A `RetryContext` has a parent context if there is a nested retry in progress in the same thread.
The parent context is occasionally useful for storing data that needs to be shared between calls to execute.
If you don't have access to the context directly, you can obtain the current context within the scope of the retries by calling `RetrySynchronizationManager.getContext()`.
By default, the context is stored in a `ThreadLocal`.
JEP 444 recommends that `ThreadLocal` should be avoided when using virtual threads, available in Java 21 and beyond.
To store the contexts in a `Map` instead of a `ThreadLocal`, call `RetrySynchronizationManager.setUseThreadLocal(false)`.
### Using `RecoveryCallback`
When a retry is exhausted, the `RetryOperations` can pass control to a different
callback: `RecoveryCallback`. To use this feature, clients can pass in the callbacks
together to the same method, as the following example shows:
```
MyObject myObject = template.execute(new RetryCallback<MyObject, Exception>() {
public MyObject doWithRetry(RetryContext context) {
// business logic here
},
new RecoveryCallback<MyObject>() {
MyObject recover(RetryContext context) throws Exception {
// recover logic here
}
});
```
If the business logic does not succeed before the template decides to abort, the client is
given the chance to do some alternate processing through the recovery callback.
## Stateless Retry
In the simplest case, a retry is just a while loop: the `RetryTemplate` can keep trying
until it either succeeds or fails. The `RetryContext` contains some state to determine
whether to retry or abort. However, this state is on the stack, and there is no need to
store it anywhere globally. Consequently, we call this "stateless retry". The distinction
between stateless and stateful retry is contained in the implementation of `RetryPolicy`
(`RetryTemplate` can handle both). In a stateless retry, the callback is always executed
in the same thread as when it failed on retry.
## Stateful Retry
Where the failure has caused a transactional resource to become invalid, there are some
special considerations. This does not apply to a simple remote call, because there is (usually) no
transactional resource, but it does sometimes apply to a database update,
especially when using Hibernate. In this case, it only makes sense to rethrow the
exception that called the failure immediately so that the transaction can roll back and
we can start a new (and valid) one.
In these cases, a stateless retry is not good enough, because the re-throw and roll back
necessarily involve leaving the `RetryOperations.execute()` method and potentially losing
the context that was on the stack. To avoid losing the context, we have to introduce a
storage strategy to lift it off the stack and put it (at a minimum) in heap storage. For
this purpose, Spring Retry provides a storage strategy called `RetryContextCache`, which
you can inject into the `RetryTemplate`. The default implementation of the
`RetryContextCache` is in-memory, using a simple `Map`. It has a strictly enforced maximum
capacity, to avoid memory leaks, but it does not have any advanced cache features (such as
time to live). You should consider injecting a `Map` that has those features if you need
them. For advanced usage with multiple processes in a clustered environment, you might
also consider implementing the `RetryContextCache` with a cluster cache of some sort
(though, even in a clustered environment, this might be overkill).
Part of the responsibility of the `RetryOperations` is to recognize the failed operations
when they come back in a new execution (and usually wrapped in a new transaction). To
facilitate this, Spring Retry provides the `RetryState` abstraction. This works in
conjunction with special `execute` methods in the `RetryOperations`.
The failed operations are recognized by identifying the state across multiple invocations
of the retry. To identify the state, you can provide a `RetryState` object that is
responsible for returning a unique key that identifies the item. The identifier is used as
a key in the `RetryContextCache`.
> *Warning:*
Be very careful with the implementation of `Object.equals()` and `Object.hashCode()` in
the key returned by `RetryState`. The best advice is to use a business key to identify the
items. In the case of a JMS message, you can use the message ID.
When the retry is exhausted, you also have the option to handle the failed item in a
different way, instead of calling the `RetryCallback` (which is now presumed to be likely
to fail). As in the stateless case, this option is provided by the `RecoveryCallback`,
which you can provide by passing it in to the `execute` method of `RetryOperations`.
The decision to retry or not is actually delegated to a regular `RetryPolicy`, so the
usual concerns about limits and timeouts can be injected there (see the [Additional Dependencies](#Additional_Dependencies) section).
## Retry Policies
Inside a `RetryTemplate`, the decision to retry or fail in the `execute` method is
determined by a `RetryPolicy`, which is also a factory for the `RetryContext`. The
`RetryTemplate` is responsible for using the current policy to create a `RetryContext` and
passing that in to the `RetryCallback` at every attempt. After a callback fails, the
`RetryTemplate` has to make a call to the `RetryPolicy` to ask it to update its state
(which is stored in `RetryContext`). It then asks the policy if another attempt can be
made. If another attempt cannot be made (for example, because a limit has been reached or
a timeout has been detected), the policy is also responsible for identifying the
exhausted state -- but not for handling the exception. `RetryTemplate` throws the
original exception, except in the stateful case, when no recovery is available. In that
case, it throws `RetryExhaustedException`. You can also set a flag in the
`RetryTemplate` to have it unconditionally throw the original exception from the
callback (that is, from user code) instead.
> *Tip:*
Failures are inherently either retryable or not -- if the same exception is always going
to be thrown from the business logic, it does not help to retry it. So you should not
retry on all exception types. Rather, try to focus on only those exceptions that you
expect to be retryable. It is not usually harmful to the business logic to retry more
aggressively, but it is wasteful, because, if a failure is deterministic, time is spent
retrying something that you know in advance is fatal.
Spring Retry provides some simple general-purpose implementations of stateless
`RetryPolicy` (for example, a `SimpleRetryPolicy`) and the `TimeoutRetryPolicy` used in
the preceding example.
The `SimpleRetryPolicy` allows a retry on any of a named list of exception types, up to a
fixed number of times. The following example shows how to use it:
```java
// Set the max attempts including the initial attempt before retrying
// and retry on all exceptions (this is the default):
SimpleRetryPolicy policy = new SimpleRetryPolicy(5, Collections.singletonMap(Exception.class, true));
// Use the policy...
RetryTemplate template = new RetryTemplate();
template.setRetryPolicy(policy);
template.execute(new RetryCallback<MyObject, Exception>() {
public MyObject doWithRetry(RetryContext context) {
// business logic here
}
});
```
A more flexible implementation called `ExceptionClassifierRetryPolicy` is also available.
It lets you configure different retry behavior for an arbitrary set of exception types
through the `ExceptionClassifier` abstraction. The policy works by calling on the
classifier to convert an exception into a delegate `RetryPolicy`. For example, one
exception type can be retried more times before failure than another, by mapping it to a
different policy.
You might need to implement your own retry policies for more customized decisions. For
instance, if there is a well-known, solution-specific, classification of exceptions into
retryable and not retryable.
## Backoff Policies
When retrying after a transient failure, it often helps to wait a bit before trying again,
because (usually) the failure is caused by some problem that can be resolved only by
waiting. If a `RetryCallback` fails, the `RetryTemplate` can pause execution according to
the `BackoffPolicy`. The following listing shows the definition of the `BackoffPolicy`
interface:
```java
public interface BackoffPolicy {
BackOffContext start(RetryContext context);
void backOff(BackOffContext backOffContext)
throws BackOffInterruptedException;
}
```
A `BackoffPolicy` is free to implement the backoff in any way it chooses. The policies
provided by Spring Retry all use `Object.wait()`. A common use case is to
back off with an exponentially increasing wait period, to avoid two retries getting into
lock step and both failing (a lesson learned from Ethernet). For this purpose, Spring
Retry provides `ExponentialBackoffPolicy`. Spring Retry also provides randomized versions
of delay policies that are quite useful to avoid resonating between related failures in a
complex system, by adding jitter.
## Listeners
It is often useful to be able to receive additional callbacks for cross-cutting concerns across a number of different retries.
For this purpose, Spring Retry provides the `RetryListener` interface.
The `RetryTemplate` lets you register `RetryListener` instances, and they are given callbacks with the `RetryContext` and `Throwable` (where available during the iteration).
The following listing shows the `RetryListener` interface:
```java
public interface RetryListener {
default <T, E extends Throwable> boolean open(RetryContext context, RetryCallback<T, E> callback) {
return true;
}
default <T, E extends Throwable> void onSuccess(RetryContext context, RetryCallback<T, E> callback, T result) {
}
default <T, E extends Throwable> void onError(RetryContext context, RetryCallback<T, E> callback,
Throwable throwable) {
}
default <T, E extends Throwable> void close(RetryContext context, RetryCallback<T, E> callback,
Throwable throwable) {
}
}
```
The `open` and `close` callbacks come before and after the entire retry in the simplest case, and `onSuccess`, `onError` apply to the individual `RetryCallback` calls; the current retry count can be obtained from the `RetryContext`.
The close method might also receive a `Throwable`.
Starting with version 2.0, the `onSuccess` method is called after a successful call to the callback.
This allows the listener to examine the result and throw an exception if the result doesn't match some expected criteria.
The type of the exception thrown is then used to determine whether the call should be retried or not, based on the retry policy.
If there has been an error, it is the last one thrown by the `RetryCallback`.
Note that when there is more than one listener, they are in a list, so there is an order.
In this case, `open` is called in the same order, while `onSuccess`, `onError`, and `close` are called in reverse order.
### Listeners for Reflective Method Invocations
When dealing with methods that are annotated with `@Retryable` or with Spring AOP intercepted methods, Spring Retry allows a detailed inspection of the method invocation within the `RetryListener` implementation.
Such a scenario could be particularly useful when there is a need to monitor how often a certain method call has been retried and expose it with detailed tagging information (such as class name, method name, or even parameter values in some exotic cases).
Starting with version 2.0, the `MethodInvocationRetryListenerSupport` has a new method `doOnSuccess`.
The following example registers such a listener:
```java
template.registerListener(new MethodInvocationRetryListenerSupport() {
@Override
protected <T, E extends Throwable> void doClose(RetryContext context,
MethodInvocationRetryCallback<T, E> callback, Throwable throwable) {
monitoringTags.put(labelTagName, callback.getLabel());
Method method = callback.getInvocation()
.getMethod();
monitoringTags.put(classTagName,
method.getDeclaringClass().getSimpleName());
monitoringTags.put(methodTagName, method.getName());
// register a monitoring counter with appropriate tags
// ...
@Override
protected <T, E extends Throwable> void doOnSuccess(RetryContext context,
MethodInvocationRetryCallback<T, E> callback, T result) {
Object[] arguments = callback.getInvocation().getArguments();
// decide whether the result for the given arguments should be accepted
// or retried according to the retry policy
}
}
});
```
## Declarative Retry
Sometimes, you want to retry some business processing every time it happens. The classic
example of this is the remote service call. Spring Retry provides an AOP interceptor that
wraps a method call in a `RetryOperations` instance for exactly this purpose. The
`RetryOperationsInterceptor` executes the intercepted method and retries on failure
according to the `RetryPolicy` in the provided `RepeatTemplate`.
### <a name="javaConfigForRetryProxies"></a> Java Configuration for Retry Proxies
You can add the `@EnableRetry` annotation to one of your `@Configuration` classes and use
`@Retryable` on the methods (or on the type level for all methods) that you want to retry.
You can also specify any number of retry listeners. The following example shows how to do
so:
```java
@Configuration
@EnableRetry
public class Application {
@Bean
public Service service() {
return new Service();
}
@Bean public RetryListener retryListener1() {
return new RetryListener() {...}
}
@Bean public RetryListener retryListener2() {
return new RetryListener() {...}
}
}
@Service
class Service {
@Retryable(RemoteAccessException.class)
public service() {
// ... do something
}
}
```
You can use the attributes of `@Retryable` to control the `RetryPolicy` and `BackoffPolicy`, as follows:
```java
@Service
class Service {
@Retryable(maxAttempts=12, backoff=@Backoff(delay=100, maxDelay=500))
public service() {
// ... do something
}
}
```
The preceding example creates a random backoff between 100 and 500 milliseconds and up to
12 attempts. There is also a `stateful` attribute (default: `false`) to control whether
the retry is stateful or not. To use stateful retry, the intercepted method has to have
arguments, since they are used to construct the cache key for the state.
The `@EnableRetry` annotation also looks for beans of type `Sleeper` and other strategies
used in the `RetryTemplate` and interceptors to control the behavior of the retry at runtime.
The `@EnableRetry` annotation creates proxies for `@Retryable` beans, and the proxies
(that is, the bean instances in the application) have the `Retryable` interface added to
them. This is purely a marker interface, but it might be useful for other tools looking to
apply retry advice (they should usually not bother if the bean already implements
`Retryable`).
If you want to take an alternative code path when
the retry is exhausted, you can supply a recovery method. Methods should be declared in the same class as the `@Retryable`
instance and marked `@Recover`. The return type must match the `@Retryable` method. The arguments
for the recovery method can optionally include the exception that was thrown and
(optionally) the arguments passed to the original retryable method (or a partial list of
them as long as none are omitted up to the last one needed). The following example shows how to do so:
```java
@Service
class Service {
@Retryable(retryFor = RemoteAccessException.class)
public void service(String str1, String str2) {
// ... do something
}
@Recover
public void recover(RemoteAccessException e, String str1, String str2) {
// ... error handling making use of original args if required
}
}
```
To resolve conflicts between multiple methods that can be picked for recovery, you can explicitly specify recovery method name.
The following example shows how to do so:
```java
@Service
class Service {
@Retryable(recover = "service1Recover", retryFor = RemoteAccessException.class)
public void service1(String str1, String str2) {
// ... do something
}
@Retryable(recover = "service2Recover", retryFor = RemoteAccessException.class)
public void service2(String str1, String str2) {
// ... do something
}
@Recover
public void service1Recover(RemoteAccessException e, String str1, String str2) {
// ... error handling making use of original args if required
}
@Recover
public void service2Recover(RemoteAccessException e, String str1, String str2) {
// ... error handling making use of original args if required
}
}
```
Version 1.3.2 and later supports matching a parameterized (generic) return type to detect the correct recovery method:
```java
@Service
class Service {
@Retryable(retryFor = RemoteAccessException.class)
public List<Thing1> service1(String str1, String str2) {
// ... do something
}
@Retryable(retryFor = RemoteAccessException.class)
public List<Thing2> service2(String str1, String str2) {
// ... do something
}
@Recover
public List<Thing1> recover1(RemoteAccessException e, String str1, String str2) {
// ... error handling for service1
}
@Recover
public List<Thing2> recover2(RemoteAccessException e, String str1, String str2) {
// ... error handling for service2
}
}
```
Version 1.2 introduced the ability to use expressions for certain properties. The
following example show how to use expressions this way:
```java
@Retryable(exceptionExpression="message.contains('this can be retried')")
public void service1() {
...
}
@Retryable(exceptionExpression="message.contains('this can be retried')")
public void service2() {
...
}
@Retryable(exceptionExpression="@exceptionChecker.shouldRetry(#root)",
maxAttemptsExpression = "#{@integerFiveBean}",
backoff = @Backoff(delayExpression = "#{1}", maxDelayExpression = "#{5}", multiplierExpression = "#{1.1}"))
public void service3() {
...
}
```
Since Spring Retry 1.2.5, for `exceptionExpression`, templated expressions (`#{...}`) are
deprecated in favor of simple expression strings
(`message.contains('this can be retried')`).
Expressions can contain property placeholders, such as `#{${max.delay}}` or
`#{@exceptionChecker.${retry.method}(#root)}`. The following rules apply:
- `exceptionExpression` is evaluated against the thrown exception as the `#root` object.
- `maxAttemptsExpression` and the `@BackOff` expression attributes are evaluated once,
during initialization. There is no root object for the evaluation but they can reference
other beans in the context
Starting with version 2.0, expressions in `@Retryable`, `@CircuitBreaker`, and `BackOff` can be evaluated once, during application initialization, or at runtime.
With earlier versions, evaluation was always performed during initialization (except for `Retryable.exceptionExpression` which is always evaluated at runtime).
When evaluating at runtime, a root object containing the method arguments is passed to the evaluation context.
**Note:** The arguments are not available until the method has been called at least once; they will be null initially, which means, for example, you can't set the initial `maxAttempts` using an argument value, you can, however, change the `maxAttempts` after the first failure and before any retries are performed.
Also, the arguments are only available when using stateless retry (which includes the `@CircuitBreaker`).
Version 2.0 adds more flexibility to exception classification.
```java
@Retryable(retryFor = RuntimeException.class, noRetryFor = IllegalStateException.class, notRecoverable = {
IllegalArgumentException.class, IllegalStateException.class })
public void service() {
...
}
@Recover
public void recover(Throwable cause) {
...
}
```
`retryFor` and `noRetryFor` are replacements of `include` and `exclude` properties, which are now deprecated.
The new `notRecoverable` property allows the recovery method(s) to be skipped, even if one matches the exception type; the exception is thrown to the caller either after retries are exhausted, or immediately, if the exception is not retryable.
##### Examples
```java
@Retryable(maxAttemptsExpression = "@runtimeConfigs.maxAttempts",
backoff = @Backoff(delayExpression = "@runtimeConfigs.initial",
maxDelayExpression = "@runtimeConfigs.max", multiplierExpression = "@runtimeConfigs.mult"))
public void service() {
...
}
```
Where `runtimeConfigs` is a bean with those properties.
```java
@Retryable(maxAttemptsExpression = "args[0] == 'something' ? 3 : 1")
public void conditional(String string) {
...
}
```
#### <a name="Additional_Dependencies"></a> Additional Dependencies
The declarative approach to applying retry handling by using the `@Retryable` annotation
shown earlier has an additional runtime dependency on AOP classes that need to be declared
in your project. If your application is implemented by using Spring Boot, this dependency
is best resolved by using the Spring Boot starter for AOP. For example, for Gradle, add
the following line to your `build.gradle` file:
```
runtimeOnly 'org.springframework.boot:spring-boot-starter-aop'
```
For non-Boot apps, you need to declare a runtime dependency on the latest version of
AspectJ's `aspectjweaver` module. For example, for Gradle, you should add the following
line to your `build.gradle` file:
```
runtimeOnly 'org.aspectj:aspectjweaver:1.9.20.1'
```
### Further customizations
Starting from version 1.3.2 and later `@Retryable` annotation can be used in custom composed annotations to create your own annotations with predefined behaviour.
For example if you discover you need two kinds of retry strategy, one for local services calls, and one for remote services calls, you could decide
to create two custom annotations `@LocalRetryable` and `@RemoteRetryable` that differs in the retry strategy as well in the maximum number of retries.
To make custom annotation composition work properly you can use `@AliasFor` annotation, for example on the `recover` method, so that you can further extend the versatility of your custom annotations and allow the `recover` argument value
to be picked up as if it was set on the `recover` method of the base `@Retryable` annotation.
Usage Example:
```java
@Service
class Service {
...
@LocalRetryable(include = TemporaryLocalException.class, recover = "service1Recovery")
public List<Thing> service1(String str1, String str2){
//... do something
}
public List<Thing> service1Recovery(TemporaryLocalException ex,String str1, String str2){
//... Error handling for service1
}
...
@RemoteRetryable(include = TemporaryRemoteException.class, recover = "service2Recovery")
public List<Thing> service2(String str1, String str2){
//... do something
}
public List<Thing> service2Recovery(TemporaryRemoteException ex, String str1, String str2){
//... Error handling for service2
}
...
}
```
```java
@Target({ ElementType.METHOD, ElementType.TYPE })
@Retention(RetentionPolicy.RUNTIME)
@Retryable(maxAttempts = "3", backoff = @Backoff(delay = "500", maxDelay = "2000", random = true)
)
public @interface LocalRetryable {
@AliasFor(annotation = Retryable.class, attribute = "recover")
String recover() default "";
@AliasFor(annotation = Retryable.class, attribute = "value")
Class<? extends Throwable>[] value() default {};
@AliasFor(annotation = Retryable.class, attribute = "include")
Class<? extends Throwable>[] include() default {};
@AliasFor(annotation = Retryable.class, attribute = "exclude")
Class<? extends Throwable>[] exclude() default {};
@AliasFor(annotation = Retryable.class, attribute = "label")
String label() default "";
}
```
```java
@Target({ ElementType.METHOD, ElementType.TYPE })
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Retryable(maxAttempts = "5", backoff = @Backoff(delay = "1000", maxDelay = "30000", multiplier = "1.2", random = true)
)
public @interface RemoteRetryable {
@AliasFor(annotation = Retryable.class, attribute = "recover")
String recover() default "";
@AliasFor(annotation = Retryable.class, attribute = "value")
Class<? extends Throwable>[] value() default {};
@AliasFor(annotation = Retryable.class, attribute = "include")
Class<? extends Throwable>[] include() default {};
@AliasFor(annotation = Retryable.class, attribute = "exclude")
Class<? extends Throwable>[] exclude() default {};
@AliasFor(annotation = Retryable.class, attribute = "label")
String label() default "";
}
```
### XML Configuration
The following example of declarative iteration uses Spring AOP to repeat a service call to
a method called `remoteCall`:
```xml
<aop:config>
<aop:pointcut id="transactional"
expression="execution(* com..*Service.remoteCall(..))" />
<aop:advisor pointcut-ref="transactional"
advice-ref="retryAdvice" order="-1"/>
</aop:config>
<bean id="retryAdvice"
class="org.springframework.retry.interceptor.RetryOperationsInterceptor"/>
```
For more detail on how to configure AOP interceptors, see the [Spring Framework Documentation](https://docs.spring.io/spring/docs/current/spring-framework-reference/index.html).
The preceding example uses a default `RetryTemplate` inside the interceptor. To change the
policies or listeners, you need only inject an instance of `RetryTemplate` into the
interceptor.
## Contributing
Spring Retry is released under the non-restrictive Apache 2.0 license
and follows a very standard Github development process, using Github
tracker for issues and merging pull requests into the main branch. If you want
to contribute even something trivial, please do not hesitate, but do please
follow the guidelines in the next paragraph.
Before we can accept a non-trivial patch or pull request, we need you
to sign the [contributor's agreement](https://cla.pivotal.io/). Signing
the contributor's agreement does not grant anyone commit rights to the
main repository, but it does mean that we can accept your
contributions, and you will get an author credit if we do. Active
contributors might be asked to join the core team and be given the
ability to merge pull requests.
## Getting Support
Check out the [Spring Retry tags on Stack Overflow](https://stackoverflow.com/questions/tagged/spring-retry). [Commercial support](https://spring.io/support) is available too.
## Code of Conduct
This project adheres to the [Contributor Covenant](https://github.com/spring-projects/spring-retry/blob/main/CODE_OF_CONDUCT.adoc).
By participating, you are expected to uphold this code. Please report unacceptable behavior to
spring-code-of-conduct@pivotal.io.
| 0 |
xwjie/PLMCodeTemplate | 给部门制定的代码框架模板 | aop log4j-mdc spring threadlocal | # 晓风轻的Spring开发代码模板
> 本文的面向目标群为编写业务代码的初学者。
>
> 本文的目的是编写简单易读的代码。
给部门制定的代码框架模板。追求工匠精神,编写简单代码。
作者微信交流
 
pdf电子书请见ebook目录。
[`SpringBoot版本在这里`](https://github.com/xwjie/ElementVueSpringbootCodeTemplate)**,持续更新中,后续加入vue+element的代码模板,欢迎加星加watch。**
# 前言
参考 [**程序员你为什么这么累**](https://zhuanlan.zhihu.com/p/28705206) 系列文章 ,里面有详细的讲解,评论里面有不同观点的讨论,建议也看看,相信对你有帮助。

# 工程使用说明
工程使用jdk6+,使用了[lombok](https://projectlombok.org/)插件。在 Idea 里面选择 `source`目录导入 `Maven` 工程即可。然后在Tomcat里面运行工程即可。
启动项目,访问地址 `http://localhost:8080/+[应用名(可为空)]` 即可。
[工程使用详细说明](docs/install.md)
# 目录
**以下为简要说明,详细说明请看 [https://xwjie.github.io/](https://xwjie.github.io/)**
> * 优雅编码 - 接口定义规范
> * 优雅编码 - ResultBean的重要性和约束
> * 优雅编码 - 异常处理
> * 优雅编码 - 参数校验和国际化规范
> * 优雅编码 - 日志打印
> * 优雅编码 - 工具类编写
> * 技术点总结
> * 对开发组长的要求
> * 对开发人员的建议
# 优雅编码 - 接口定义规范
请阅读 [我的编码习惯 - 接口定义](https://zhuanlan.zhihu.com/p/28708259) ,不要犯里面的错误。
1. 所有接口都必须返回ResultBean。
2. 一开始就要考虑成功和失败二种场景。
3. ResultBean只允许出现在controller,不允许到处传。
4. 不要出现 map ,json 等复杂对象做为输入和输出。
5. 不要出现 local ,messagesource 等和业务无关的参数。
# 优雅编码 - ResultBean的重要性和约束
请阅读 [我的编码习惯 - Controller规范](https://zhuanlan.zhihu.com/p/28717374)。
这里都是对开发组长的要求。使用 `AOP` 统一处理异常,并根据不同异常返回不同返回码。尤其关注非用户自己抛出的异常。
# 优雅编码 - 异常处理
请阅读 [我的编码习惯 - 异常处理](https://zhuanlan.zhihu.com/p/29005176)。
1. 开发人员不准捕获异常,直接抛出。
2. 少加空判断。如果对象不应该为空,就不需要加空判断,加了空判断就要测试为空和不为空二种情况。
其他对开发组长的要求请见上面的文章和代码。
# 优雅编码 - 日志打印
请阅读 [我的编程习惯 - 日志建议](https://zhuanlan.zhihu.com/p/28629319)。
## 分支语句的变量需要打印变量
分支语句变量会影响代码走向,不打日志无法知道究竟走那个分支。如下
```Java
// optype决定代码走向,需要打印日志
logger.info("edit user, opType:" + opType);
if (opType == CREATE) {
// 新增操作
} else if (opType == UPDATE) {
// 修改操作
} else {
// 错误的类型,抛出异常
throw new IllegalArgumentException("unknown optype:" + opType);
}
```
如果没有打印optype的值,出了问题你只能从前找到后,看optype是什么了,很浪费时间。
> 重要建议:养成增加else语句,把不合法参数抛出异常的好习惯。
>
> 抛异常的时候把对应的非法值抛出来。
## 修改操作需要打印操作的对象
这点是为了跟踪。防止出现,一个数据被删除了,找不到谁做的。如
```Java
private void deleteDoc(long id) {
logger.info("delete doc, id:" + id);
// 删除代码
}
```
## 大量数据操作的时候需要打印数据长度
建议前后打印日志,而且要打印出数据长度,目的是为了知道 `处理了多少数据用了多少时间` 。如一个操作用了3秒钟,性能是好还是坏? 如果处理了1条数据,那么可能就是性能差,如果处理了10000条数据,那么可能就是性能好。
```Java
logger.info("query docment start, params:" + params);
List<Document> docList = query(params);
logger.info("query docment done, size:" + docList.size())
```
## 使用log4j的MDC打印用户名等额外信息
有时候,业务量大的系统要找到某一个用户的操作日志定位问题非常痛苦,每一个日志上加用户名又低效也容易漏掉,所以我们要在更高层级上解决这些共性问题。
我们使用log4j的 `MDC` 功能达成这个目的。在用户登录后,把用户标志放到 `MDC` 中。
```Java
private final static ThreadLocal<String> tlUser = new ThreadLocal<>();
public static final String KEY_USER = "user";
public static void setUser(String userid) {
tlUser.set(userid);
// 把用户信息放到log4j
MDC.put(KEY_USER, userid);
}
```
然后修改log4j配置,pattern上增加 `%X{user}` ,位置随意。增加线程相关配置 `[%t]` 。更多参数[log4j变量](https://logging.apache.org/log4j/1.2/apidocs/org/apache/log4j/PatternLayout.html)
```XML
<layout class="org.apache.log4j.PatternLayout">
<param name="ConversionPattern" value="[%t]%-d{MM-dd HH:mm:ss,SSS} %-5p: %X{user} - %c - %m%n" />/>
</layout>
```
最终效果图:

> 没有用户信息的时候并不会报错,而是空串。
>
> 不要一开始就关注日志级别和日志性能,规则越多越难落地。
>
> 必须记得清空。
## 集群环境下需要在静态服务器增加配置,返回处理机器的信息到响应头
配置后可以直接找到代码在那个机器上运行,快速定位。举例,`Nginx` 集群最简配置(主要使用 `$upstream_addr`) :
```
upstream code_server{
server 127.0.0.1:8080;
server 127.0.0.1:18080;
ip_hash;
keepalive 32;
}
server{
listen 80;
server_name xwjie.com;
location /plm {
proxy_pass http://code_server/plm;
add_header x-slave $upstream_addr;
}
}
```
效果图:

# 优雅编码 - 参数校验和国际化规范
请阅读 [我的编码习惯 - 参数校验和国际化规范](https://zhuanlan.zhihu.com/p/29129469) 。
1. 调用自己的校验函数
2. 业务代码任何地方参数上都不要出现local,messagesource
3. 异常信息里面,不正确的参数值要提示出来,减少定位时间
4. 国际化参数不要放到每一个url上
# 优雅编码 - 工具类编写
请阅读 [我的编码习惯 - 工具类规范](https://zhuanlan.zhihu.com/p/29199049) 。
工具类的目标是要编写通用灵活的方法。重点注意参数的优化,多使用重载。
1. 隐藏实现,编写自己的工具类方法,不要再业务代码直接调用第三方工具类
2. 使用父类/接口
3. 使用重载编写衍生函数组
4. 使用静态引入,方便找到工具方法
5. 物理上独立存放,独立维护
# 技术点总结
1. spring的aop的使用
2. log4j的MDC使用
3. JDK的ThreadLocal的使用
# 对开发组长的要求
定义好代码框架,不要做太多、太细的要求,否则无法落地。这篇文章中,规范中要求做到的少,不准做的多,落地相对容易。
1. 定义好统一的接口格式、异常、常量等
2. 多使用AOP和ThreadLocal简化代码
3. 亲自编写校验函数和工具类
4. 代码评审中,严格控制函数的参数,不允许出现复杂参数和业务无关的参数
# 对开发人员的建议
1. 不要养成面对debug编程,用日志代替debug
2. 不要一上来就做整个功能测试,要一行一行代码一个一个函数测试
3. 谨慎捕获异常和加空判断,加了空判断就要测试为空和不为空二种情况
4. 日志也是代码的一部分,提交代码前先运行看一遍操作日志
| 0 |
java-deobfuscator/deobfuscator | The real deal | null | # Deobfuscator
This project aims to deobfuscate most commercially-available obfuscators for Java.
## Updates
To download an updated version of Java Deobfuscator, go to the releases tab.
If you would like to run this program with a GUI, go to https://github.com/java-deobfuscator/deobfuscator-gui and grab a download. Put the deobfuscator-gui.jar in the same folder as deobfuscator.jar.
## Quick Start
* [Download](https://github.com/java-deobfuscator/deobfuscator/releases) the deobfuscator. The latest build is recommended.
* If you know what obfuscators were used, skip the next two steps
* Create `detect.yml` with the following contents. Replace `input.jar` with the name of the input
```yaml
input: input.jar
detect: true
```
* Run `java -jar deobfuscator.jar --config detect.yml` to determine the obfuscators used
* Create `config.yml` with the following contents. Replace `input.jar` with the name of the input
```yaml
input: input.jar
output: output.jar
transformers:
- [fully-qualified-name-of-transformer]
- [fully-qualified-name-of-transformer]
- [fully-qualified-name-of-transformer]
- ... etc
```
* Run `java -jar deobfuscator.jar`
* Re-run the detection if the JAR was not fully deobfuscated - it's possible to layer obfuscations
Take a look at [USAGE.md](USAGE.md) or [wiki](https://github.com/java-deobfuscator/deobfuscator/wiki) for more information.
## It didn't work
If you're trying to recover the names of classes or methods, tough luck. That information is typically stripped out and there's no way to recover it.
If you are using one of our transformers, check out the [commonerrors](commonerrors) folder to check for tips.
Otherwise, check out [this guide](CUSTOMTRANSFORMER.md) on how to implement your own transformer (also, open a issue/PR so I can add support for it)
## Supported Obfuscators
* [Zelix Klassmaster](http://www.zelix.com/)
* [Stringer](https://jfxstore.com/stringer/)
* [Allatori](http://www.allatori.com/)
* [DashO](https://www.preemptive.com/products/dasho/overview)
* [DexGuard](https://www.guardsquare.com/dexguard)
* [ClassGuard](https://www.zenofx.com/classguard/)
* Smoke (dead, website archive [here](https://web.archive.org/web/20170918112921/https://newtownia.net/smoke/))
* SkidSuite2 (dead, archive [here](https://github.com/GenericException/SkidSuite/tree/master/archive/skidsuite-2))
## List of Transformers
The automagic detection should be able to recommend the transformers you'll need to use. However, it may not be up to date. If you're familiar with Java reverse engineering, feel free to [take a look around](src/main/java/com/javadeobfuscator/deobfuscator/transformers) and use what you need.
## FAQs
#### I got an error that says "Could not locate a class file"
You need to specify all the JARs that the input file references. You'll almost always need to add `rt.jar`
(which contains all the classes used by the Java Runtime)
#### I got an error that says "A StackOverflowError occurred during deobfuscation"
Increase your stack size. For example, `java -Xss128m -jar deobfuscator.jar`
#### Does this work on Android apps?
Technically, yes, you could use something like [dex2jar](https://github.com/pxb1988/dex2jar)
or [enjarify](https://github.com/storyyeller/enjarify). However, dex -> jar conversion is lossy at best.
Try [simplify](https://github.com/CalebFenton/simplify) or [dex-oracle](https://github.com/CalebFenton/dex-oracle) first.
They were written specifically for Android apps.
## Licensing
Java Deobfuscator is licensed under the Apache 2.0 license.
| 0 |
spinnaker/kayenta | Automated Canary Service | hacktoberfest | Kayenta
====
[](https://travis-ci.org/spinnaker/kayenta)
Kayenta is a platform for Automated Canary Analysis (ACA). It is used by Spinnaker to enable automated canary deployments. Please see the comprehensive [canary documentation](https://www.spinnaker.io/guides/user/canary/stage/) for more details.
### Canary Release
A canary release is a technique to reduce the risk from deploying a new version of software into production. A new version of software, referred to as the canary, is deployed to a small subset of users alongside the stable running version. Traffic is split between these two versions such that a portion of incoming requests are diverted to the canary. This approach can quickly uncover any problems with the new version without impacting the majority of users.
The quality of the canary version is assessed by comparing key metrics that describe the behavior of the old and new versions. If there is significant degradation in these metrics, the canary is aborted and all of the traffic is routed to the stable version in an effort to minimize the impact of unexpected behavior.
Canaries are usually run against deployments containing changes to code, but they
can also be used for operational changes, including changes to configuration.
### Frequently Asked Questions
See the [FAQ](docs/faq.md).
### Creating Canary Config
See the [Canary Config Object model](docs/canary-config.md) for how a canary config is defined in [Markdown Syntax for Object Notation (MSON)](https://github.com/apiaryio/mson).
### Debugging
To start the JVM in debug mode, set the Java system property `DEBUG=true`:
```
./gradlew -DDEBUG=true
```
The JVM will then listen for a debugger to be attached on port 8191. The JVM will _not_ wait for the debugger
to be attached before starting Kayenta; the relevant JVM arguments can be seen and modified as needed in `build.gradle`.
### Running Standalone Kayenta Locally
You can run a standalone kayenta instance locally with `docker-compose`.
```
# Copy and edit example config accordingly
cp kayenta-web/config/kayenta.yml ~/.spinnaker/kayenta.yml
# Build/Start Kayenta
docker-compose up
```
You should then be able to access your local kayenta instance at http://localhost:8090/swagger-ui/index.html.
| 0 |
jdbi/jdbi | The Jdbi library provides convenient, idiomatic access to relational databases in Java and other JVM technologies such as Kotlin, Clojure or Scala. | database hacktoberfest java java11 jdbc jdbi kotlin sql | 
[](https://github.com/jdbi/jdbi/actions/workflows/cd.yml) |
[](https://github.com/jdbi/jdbi/actions/workflows/ci.yml) | [](https://sonarcloud.io/summary/new_code?id=jdbi_jdbi) | [](https://sonarcloud.io/summary/new_code?id=jdbi_jdbi) | [](https://sonarcloud.io/summary/new_code?id=jdbi_jdbi)
The Jdbi library provides convenient, idiomatic access to relational databases in Java and other JVM technologies such as Kotlin, Clojure or Scala.
Jdbi is built on top of JDBC. If your database has a JDBC driver, you can use Jdbi with it.
* [Developer Guide](https://jdbi.org/)
* [Javadoc](https://jdbi.org/apidocs/)
* [User forums](https://github.com/jdbi/jdbi/discussions)
* [Mailing List](http://groups.google.com/group/jdbi)
* [Stack Overflow](https://stackoverflow.com/questions/tagged/jdbi)
Also check out the code examples in the [Examples](https://github.com/jdbi/jdbi/tree/master/examples) module.
## Acknowledgements and Funding
* <img src="docs/src/adoc/images/spotify_logo.svg" alt="spotify logo" title="spotify logo" width="30" height="30" style="vertical-align: middle; padding: 2px;"> <a href="https://engineering.atspotify.com/2023/10/announcing-the-recipients-of-the-2023-spotify-foss-fund/">Jdbi is a recipient of the Spotify FOSS 2023 Fund</a>
* <img src="docs/src/adoc/images/tidelift_logo.png" alt="tidelift logo" title="tidelift logo" width="30" height="30" style="vertical-align: middle; padding: 2px;"><a href="https://tidelift.com/funding/github/maven/org.jdbi:jdbi3-core">Jdbi is supported by Tidelift</a>
## Prerequisites
Jdbi requires Java 11 or better to run.
We run CI tests against Java 11, 17 and 21.
### Compatibility with older Java versions
Java 8, 9 and 10 are supported by any Jdbi version before **3.40.0**.
## Building
Jdbi requires a JDK version 17 or better to build. We enforce the latest LTS (currently Java 21) for releases.
Jdbi is "batteries included" and uses the [Apache Maven Wrapper](https://maven.apache.org/wrapper/). If an external Maven installation is used, Apache Maven 3.9 or later is required. Using the `make` targets requires GNU make.
All build tasks are organized as `make` targets.
Build the code an install it into the local repository:
```bash
$ make install
```
Running `make` or `make help` displays all available build targets with a short explanation. Some of the goals will require project membership privileges. The [CONTRIBUTING.md](https://github.com/jdbi/jdbi/blob/master/CONTRIBUTING.md) document contains a list of all supported targets.
To add command line parameters to the maven executions from the Makefile, set the `MAVEN_CONFIG` variable:
``` bash
% MAVEN_CONFIG="-B -fae" make install
```
## Testing
Running `make tests` runs all unit and integration tests.
Some tests use Postgres and H2 databases (the tests will spin up temporary database servers as needed). Most modern OS (Windows, MacOS, Linux) and host architecture (x86_64, aarch64) should work.
### Docker requirements
For a full release build, docker or a docker compatible environment
must be available. A small number of tests use testcontainers which in
turn requires docker.
`make install-nodocker` skips the tests when building and installing Jdbi locally. `make tests-nodocker` skips the tests when only running tests.
Supported configurations are
* Docker Desktop on MacOS
* docker-ce on Linux
* podman 3 or better on Linux and MacOS
Other docker installations such as [Colima](https://github.com/abiosoft/colima) may work but are untested and unsupported.
For podman on Linux, the podman socket must be activated (see
https://stackoverflow.com/questions/71549856/testcontainers-with-podman-in-java-tests)
for details. SELinux sometimes interferes with testcontainers if
SELinux is active; make sure that there is an exception configured.
For podman on MacOS, it is necessary to set the `DOCKER_HOST` environment variable correctly.
## Contributing
Please read
[CONTRIBUTING.md](https://github.com/jdbi/jdbi/blob/master/CONTRIBUTING.md)
for instructions to set up your development environment to build Jdbi.
## Versioning
Jdbi uses [SemVer](http://semver.org/) to version its public API.
## License
This project is licensed under the
[Apache 2.0 license](https://www.apache.org/licenses/LICENSE-2.0.html).
## Project Members
* **Brian McCallister (@brianm)** - Project Founder
* **Steven Schlansker (@stevenschlansker)**
* **Henning Schmiedehausen (@hgschmie)**
* **Matthew Hall (@qualidafial)**
* **Artem Prigoda (@arteam)**
* **Marnick L'Eau (@TheRealMarnes)**
## Special Thanks
* **Alex Harin ([@aharin](https://github.com/aharin))** - Kotlin plugins.
* **Ali Shakiba ([@shakiba](https://github.com/shakiba))** - JPA plugin
* **[@alwins0n](https://github.com/alwins0n)** - Vavr plugin.
* **Fred Deschenes ([@FredDeschenes](https://github.com/FredDeschenes))** -
Kotlin unchecked extensions for `Jdbi` functions. `@BindFields`,
`@BindMethods` annotations.
| 0 |
ctripcorp/C-OCR | C-OCR是携程自研的OCR项目,主要包括身份证、护照、火车票、签证等旅游相关证件、材料的识别。 项目包含4个部分,拒识、检测、识别、后处理。 | null | C-OCR是携程自研的OCR项目,主要包括身份证、护照、火车票、签证等旅游相关证件、材料的识别。
项目包含4个部分,拒识、检测、识别、后处理。
| 0 |
benfry/processing4 | Processing 4.x releases for Java 17 | null | This repository contains the source code for the [Processing](https://processing.org/) project for people who want to help improve the code.
If you're interested in *using* Processing, get started at the [download](https://processing.org/download) page, or read more about the project at the [home page](https://processing.org/). There are also several [tutorials](https://processing.org/tutorials) that provide a helpful introduction. They are complemented by hundreds of examples that are included with the software itself.
# Processing 4.0
Processing 4 has [important updates](https://github.com/processing/processing4/wiki/Changes-in-4.0) that prepare the platform for its future. Most significantly, this includes the move to Java 17 as well as major changes to the range of platforms we support (Apple Silicon! Raspberry Pi on 32- and 64-bit ARM!)
With any luck, many changes should be transparent to most users, in spite of how much is updated behind the scenes. More immediately visible changes include major work on the UI, including “themes” and the ability to change how sketches are named by default.
## Building the Code
[Instructions on how to build the code](https://github.com/processing/processing4/blob/master/build/README.md) are found in a README inside the `build` folder.
We've also moved to a new repository for this release so that we could cull a lot of the accumulated mess of the last 20 years, which makes `git clone` (and most other `git` operations) a lot faster.
The work on 4.0 was done by a [tiny number of people](https://github.com/processing/processing4/graphs/contributors?from=2019-10-01&to=2022-08-09&type=c) who continue working on it, unpaid, because they care about it. Please help!
## API and Internal Changes
As with all releases, we'll do [everything possible](https://twitter.com/ben_fry/status/1426282574683516928) to avoid breaking API. However, there will still be tweaks that have to be made. We'll try to keep them minor. Our goal is stability, and keeping everyone's code running.
The full list of changes can be seen in [the release notes for each version](https://github.com/processing/processing4/blob/master/build/shared/revisions.md). <b>The list below only covers changes for developers working on this repository, or that may have an impact on Library, Mode, or Tool development.</b>
### Beta 9
* Major changes to themes and some libraries too. Also changed the default branch. If you have an older checkout, do this:
git pull
git checkout main
ant clean
ant clean-libs
ant run
…or just do a fresh `git clone` and pull down the latest.
* Apple Silicon support should be complete, as far as we know. If you find otherwise, file an issue.
* Check out the long [revisions](https://github.com/processing/processing4/blob/master/build/shared/revisions.md) update for this one. Too much to cover here.
* Now using Java 17.0.4+8 from [Adoptium](https://adoptium.net/).
### Beta 8
* [Renamed](https://github.com/processing/processing4/commit/409163986ff2ff4d2dbf69c79c7eced45950d1d0) the Add Mode, Add Library, and Add Tool menu items to Manage Modes, Manage Libraries, and Manage Tools. This will require translation updates for the new text:
* `toolbar.add_mode = Add Mode...` has been replaced with `toolbar.manage_modes = Manage Modes…`
* `menu.library.add_library = Add Library...` → `menu.library.manage_libraries = Manage Libraries…`
* `menu.tools.add_tool = Add Tool...` → `menu.tools.manage_tools = Manage Tools…`
### Beta 6
* Major rewrite of `handleOpen()`, now possible to use something besides the folder name for the main sketch file (see `revisions.md` for details).
* Now using Java 17.0.2+8 from [Adoptium](https://adoptium.net/).
### Beta 3
* Now using JDK 17.0.1 and JavaFX 17.0.1.
* Major changes to `theme.txt` and theme handling in general. Now rendering toolbar icons from SVG images. More documentation later.
* Made `DrawListener` public in `PSurfaceJOGL`.
### Beta 2
* Added a workaround so that `color` can be part of package names, which gets some older code (i.e. toxiclibs) running again.
### Beta 1
* Now using JDK 11.0.12+7.
### Alpha 6
* `Editor.applyPreferences()` was `protected`, now it's `public`.
* Removed `Editor.repaintErrorBar()` and `Editor.showConsole()`. Does not appear to be in use anywhere, easy to add back if we hear otherwise.
* Renamed `TextAreaPainter.getCompositionTextpainter()` to `getCompositionTextPainter()`. This was an internal function and inconsistent with the rest of the function naming.
### Alpha 5
* ~~Known bug: code completion is currently broken. Any updates will be posted [here](https://github.com/processing/processing4/issues/177).~~ Fixed in alpha 6.
* Moved from the 11.0.2 LTS version of JavaFX to the in-progress version 16. This fixes a [garbled text](https://bugs.openjdk.java.net/browse/JDK-8234916) issue that was breaking Tools that used JavaFX.
* The minimum system version for macOS (for the PDE and exported applications) is now set to 10.14.6 (the last update of Mojave). 10.13 (High Sierra) is no longer supported by Apple as of September or December 2020 (depending on what you read), and for our sanity, we're dropping it as well.
* JavaFX has been moved out of core and into a separate library. After Java 8, it's no longer part of the JDK, so it requires additional files that were formerly included by default. The Processing version of that library comes in at 180 MB, which seems excessive to include with every project, regardless of whether it's used. (And that's not including the full Webkit implementation, which adds \~80 MB per platform.)
### Alpha 4
* `EditorState(List<Editor> editors)` changed to `EditorState.nextEditor(List<Editor> editors)`, reflecting its nature as closer to a factory method (that makes use of the Editor list) than a constructor that will also be storing information about the list of Editor objects in the created object.
### Alpha 3
* `export.embed_java.for` changed to `export.include_java` which also embeds a string for the platform for better localization support.
### Alpha 2
* ~~The minimum system version for macOS (for the PDE and exported applications) is now set to 10.13.6 (the last update of High Sierra). Apple will likely be dropping support for High Sierra in late 2020, so we may make the minimum 10.14.x by the time 4.x ships.~~
* ~~See `revisions.md` if you're using `surface.setResizable()` with this release on macOS and with P2D or P3D renderers.~~
* The `static` versions of `selectInput()`, `selectOutput()`, and `selectFolder()` in `PApplet` have been removed. These were not documented, hopefully they were not in use anyway.
* The `frame` object has been removed from `PApplet`. We've been warning folks to use `surface` since 2015, but maybe we can provide an [easy way](https://github.com/processing/processing4/issues/59) to update code from inside the PDE.
* `PImage.checkAlpha()` is now `public` instead of `protected`
* All AWT calls have been moved out of `PImage`, which may be a problem for anything that was relying on those internals
* ~~For instance, `new PImage(java.awt.Image)` is no longer available. It was an undocumented method that was `public` only because it was required by subclasses.~~ As of alpha 4, this is back, because it wasn't deprecated in 3.x, and is likely to break too many things.
* Removed `MouseEvent.getClickCount()` and `MouseEvent.getAmount()`. These had been deprecated, not clear they were used anywhere.
### Alpha 1
* `Base.defaultFileMenu` is now `protected` instead of `static public`
* Processing 4 is 64-bit only. This is the overwhelming majority of users, and we don't have the necessary help to maintain and support 32-bit systems.
| 0 |
py4j/py4j | Py4J enables Python programs to dynamically access arbitrary Java objects | distributed-systems java programming-languages python | null | 0 |
frogermcs/InstaMaterial | Implementation of Instagram with Material Design (originally based on Emmanuel Pacamalan's concept) | null | InstaMaterial
=============
### Updated
Current source code contains UI elements from [Design Support Library](http://android-developers.blogspot.com/2015/05/android-design-support-library.html). If you still want to see how custom implementations of e.g. Floating Action Button or Navigation drawer work, just checkout this source code on tag [Post 8](https://github.com/frogermcs/InstaMaterial/tree/Post-8).
Source code for implementation of Instagram with Material Design (based on Emmanuel Pacamalan's concept).
[INSTAGRAM with Material Design](https://www.youtube.com/watch?v=ojwdmgmdR_Q) concept video
[](https://android-arsenal.com/details/3/1462)
## Summary
The summary of making of InstaMaterial project is available on blog: [Instagram with Material Design concept is getting real](http://frogermcs.github.io/Instagram-with-Material-Design-concept-is-getting-real-the-summary/)
### Current build
The most recent app version is available [here](https://github.com/frogermcs/frogermcs.github.io/raw/master/files/10/InstaMaterial-release-1.0.1-2.apk)
### Full showcase of application
[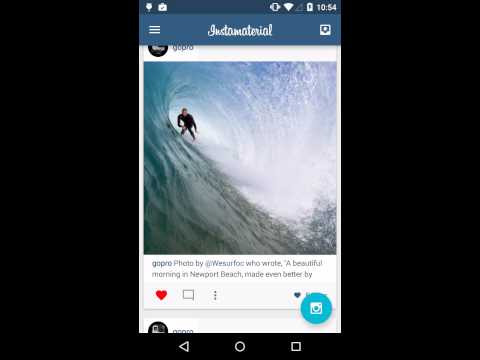](http://www.youtube.com/watch?v=VpLP__Vupxw)
## Blog posts
[Getting started - opening the app](http://frogermcs.github.io/Instagram-with-Material-Design-concept-is-getting-real)
Implemented elements and effects:
* Application intro transitions
* Toolbar
* Floating action button
* RecyclerView
[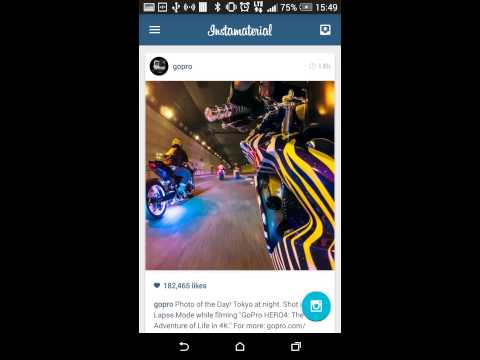](http://www.youtube.com/watch?v=fYhpc1LddHE)
---
[Comments transition](http://frogermcs.github.io/Instagram-with-Material-Design-concept-part-2-Comments-transition/)
Implemented elements and effects:
* Comments view enter and exit transition
[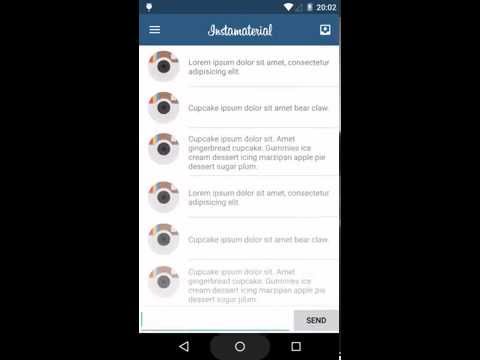](http://www.youtube.com/watch?v=b8OOaluag-w)
---
[Feed and comment buttons](http://frogermcs.github.io/InstaMaterial-concept-part-3-feed-and-comments-buttons/)
Implemented elements and effects:
* Send button animation in comments view
* ViewAnimator
* Ripples
* RecyclerView smoothness
[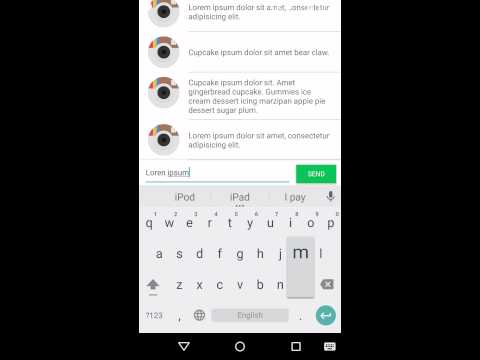](http://www.youtube.com/watch?v=GWKiN3la_CQ)
---
[Feed context menu](http://frogermcs.github.io/InstaMaterial-concept-part-4-feed-context-menu/)
Implemented elements and effects:
* Floating context menu for feed item
[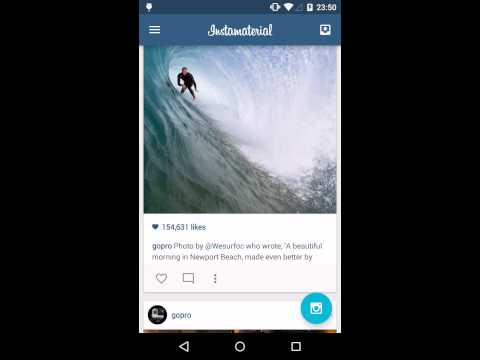](http://www.youtube.com/watch?v=eQwFwJ4Glyc)
---
[Like action effects](http://frogermcs.github.io/InstaMaterial-concept-part-5-like_action_effects/)
Implemented elements and effects:
* Like counter
* Like button animation
* Like photo animation
* AnimatorSet
* ObjectAnimator
* TextSwitcher
[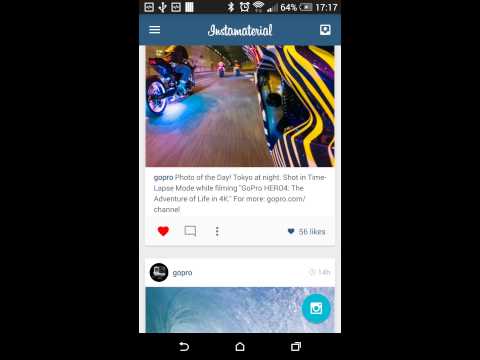](http://www.youtube.com/watch?v=KbJQ99EY5Yk)
---
[User profile](http://frogermcs.github.io/InstaMaterial-concept-part-6-user-profile/)
Implemented elements and effects:
* User profile
* Circural user photo
* Circural reveal transition
* ViewPropertyAnimator
[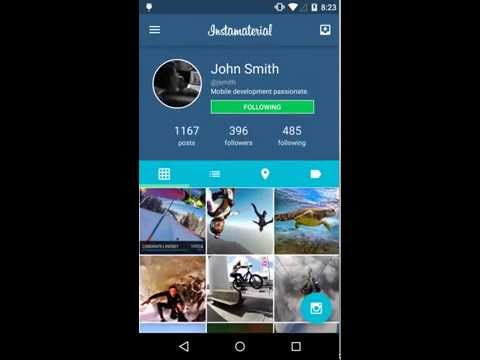](http://www.youtube.com/watch?v=EmQM0B6QPac)
---
[Navigation Drawer](http://frogermcs.github.io/InstaMaterial-concept-part-7-navigation-drawer/)
Implemented elements and effects:
* Navigation Drawer
* DrawerLayoutIstaller
[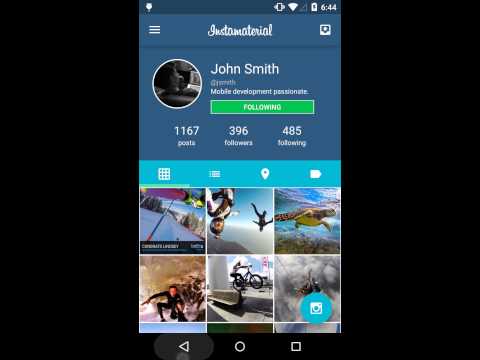](http://www.youtube.com/watch?v=rRYN1le1-ZM)
---
[Capturing photo](http://frogermcs.github.io/InstaMaterial-concept-part-8-capturing-photo/)
Implemented elements and effects:
* Camera preview
* Capturing photo
* Circular reveal
[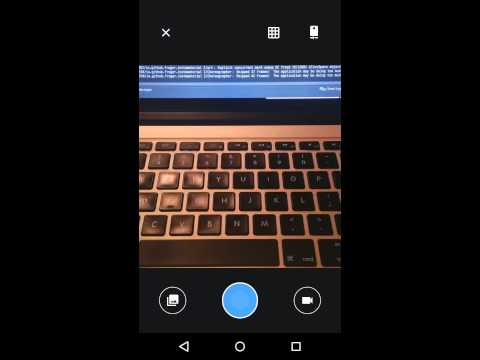](http://www.youtube.com/watch?v=0w3lGJIISTo)
---
[Publishing photo](http://frogermcs.github.io/InstaMaterial-concept-part-9-photo-publishing/)
Implemented elements and effects:
* Custom view drawing
* Intent flags
[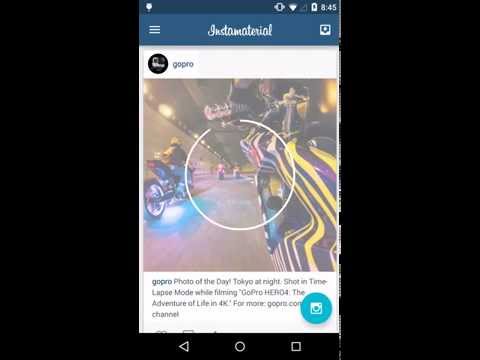](http://www.youtube.com/watch?v=YgvE3cl34ps)
| 0 |
JetBrains/xodus | Transactional schema-less embedded database used by JetBrains YouTrack and JetBrains Hub. | database db embedded-database entity-store java key-value kotlin log-structured nosql schema-less snapshot-isolation transactional xodus youtrack | # <a href="https://github.com/JetBrains/xodus/wiki"><img src="https://raw.githubusercontent.com/wiki/jetbrains/xodus/xodus.png" width=160></a>
[](https://confluence.jetbrains.com/display/ALL/JetBrains+on+GitHub)
[](https://search.maven.org/#search%7Cga%7C1%7Corg.jetbrains.xodus%20-dnq%20-time)
[](https://github.com/jetbrains/xodus/releases/latest)
[](https://www.apache.org/licenses/LICENSE-2.0.html)

[](https://stackoverflow.com/questions/tagged/xodus)
JetBrains Xodus is a transactional schema-less embedded database that is written in Java and [Kotlin](https://kotlinlang.org).
It was initially developed for [JetBrains YouTrack](https://jetbrains.com/youtrack), an issue tracking and project
management tool. Xodus is also used in [JetBrains Hub](https://jetbrains.com/hub), the user management platform
for JetBrains' team tools, and in some internal JetBrains projects.
- Xodus is transactional and fully ACID-compliant.
- Xodus is highly concurrent. Reads are completely non-blocking due to [MVCC](https://en.wikipedia.org/wiki/Multiversion_concurrency_control) and
true [snapshot isolation](https://en.wikipedia.org/wiki/Snapshot_isolation).
- Xodus is schema-less and agile. It does not require schema migrations or refactorings.
- Xodus is embedded. It does not require installation or administration.
- Xodus is written in pure Java and [Kotlin](https://kotlinlang.org).
- Xodus is free and licensed under [Apache 2.0](https://www.apache.org/licenses/LICENSE-2.0.html).
## Hello Worlds!
To start using Xodus, define dependencies:
```xml
<!-- in Maven project -->
<dependency>
<groupId>org.jetbrains.xodus</groupId>
<artifactId>xodus-openAPI</artifactId>
<version>2.0.1</version>
</dependency>
```
```kotlin
// in Gradle project
dependencies {
implementation("org.jetbrains.xodus:xodus-openAPI:2.0.1")
}
```
Read more about [managing dependencies](https://github.com/JetBrains/xodus/wiki/Managing-Dependencies).
There are two different ways to deal with data, which results in two different API layers: [Environments](https://github.com/JetBrains/xodus/wiki/Environments) and [Entity Stores](https://github.com/JetBrains/xodus/wiki/Entity-Stores).
### Environments
Add dependency on `org.jetbrains.xodus:xodus-environment:2.0.1`.
```java
try (Environment env = Environments.newInstance("/home/me/.myAppData")) {
env.executeInTransaction(txn -> {
final Store store = env.openStore("Messages", StoreConfig.WITHOUT_DUPLICATES, txn);
store.put(txn, StringBinding.stringToEntry("Hello"), StringBinding.stringToEntry("World!"));
});
}
```
### Entity Stores
Add dependency on `org.jetbrains.xodus:xodus-entity-store:2.0.1`, `org.jetbrains.xodus:xodus-environment:2.0.1` and `org.jetbrains.xodus:xodus-vfs:2.0.1`.
```java
try (PersistentEntityStore entityStore = PersistentEntityStores.newInstance("/home/me/.myAppData")) {
entityStore.executeInTransaction(txn -> {
final Entity message = txn.newEntity("Message");
message.setProperty("hello", "World!");
});
}
```
## Building from Source
[Gradle](https://www.gradle.org) is used to build, test, and publish. JDK 1.8 or higher is required. To build the project, run:
./gradlew build
To assemble JARs and skip running tests, run:
./gradlew assemble
## Find out More
- [Xodus wiki](https://github.com/JetBrains/xodus/wiki)
- [Report an issue](https://youtrack.jetbrains.com/issues/XD)
| 0 |
fusesource/jansi | Jansi is a small java library that allows you to use ANSI escape sequences to format your console output which works even on windows. | null | null | 0 |
liu-xiao-dong/JD-Test | 仿京东app 全新组件化架构升级 | arouter dagger2 fresco mvp retrofit rxjava | # JD-Test
仿京东app 采用组件化架构 屏幕适配方案可以较好解决多分辨率及同分辨率不同dpi适配;
全新组件化架构升级,相比之前的方案模块间更为解耦且使用更为方便;
### 声明 : 本项目资源采用抓包获取,仅供学习交流使用 。
### apk安装 :
[https://github.com/liu-xiao-dong/JD-Test/raw/master/app/app-release.apk](https://github.com/liu-xiao-dong/JD-Test/raw/master/app/app-release.apk)
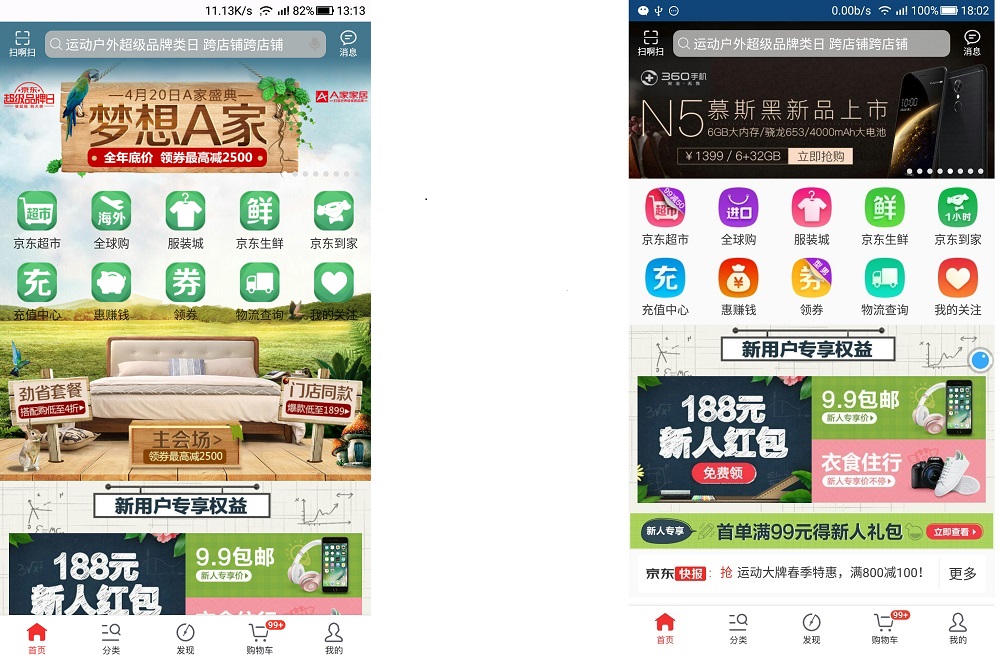
### Specs
[](https://img.shields.io/badge/API-12%2B-blue.svg?style=flat) [](https://opensource.org/licenses/Apache-2.0)
本项目为仿京东项目,资源为抓包获取,项目框架采用路由框架 ARouter 进行模块间通讯,以功能模块进行划分的组件化开发 ,模块内部采用参考google开源的mvp架构 ,
核心框架 包含 retrofit 、rxjava 、dagger2 、fresco 以及个人开源的诸多优秀项目;当然现成的轮子也有不合适的地方,在这些轮子的基础上修改以及自己造轮子组成了
现有的项目,这套架构也是我应用与项目中的架构,后期也会不断扩展维护 ,欢迎大家提issues ,喜欢就直接拿去用 ,绝不收取任何费用(好吧 , 想收也没人给 ^-^!) 。
话说一切没有gif图的项目都是耍流氓,上图:
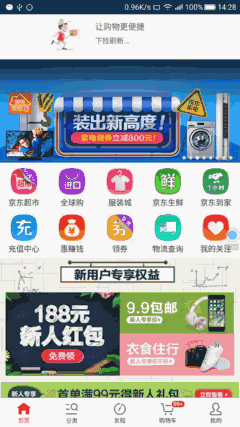
***
再来几张非主流分辨率截图
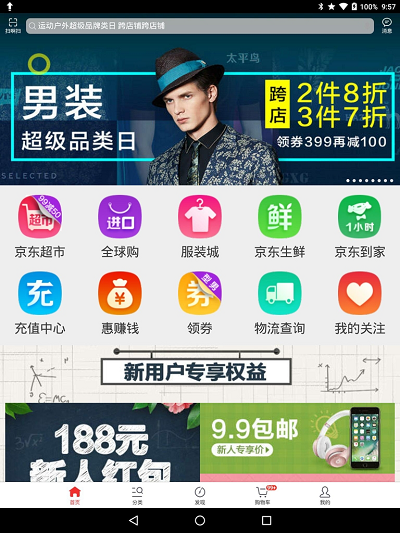 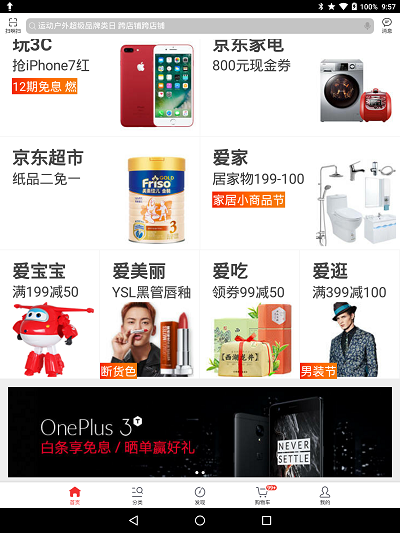
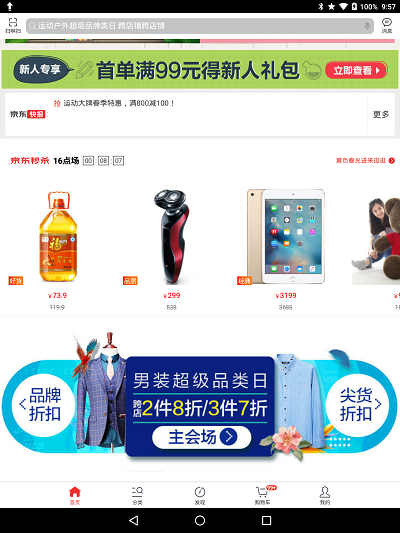 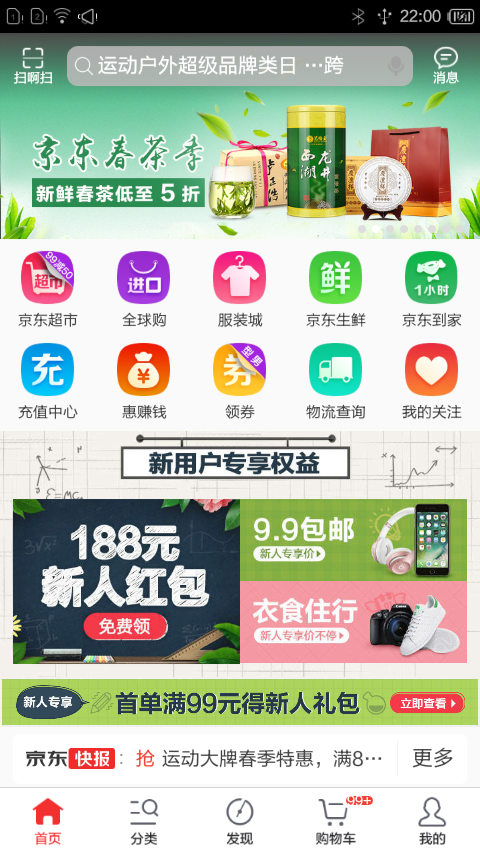
项目架构如下图:
# 旧的架构
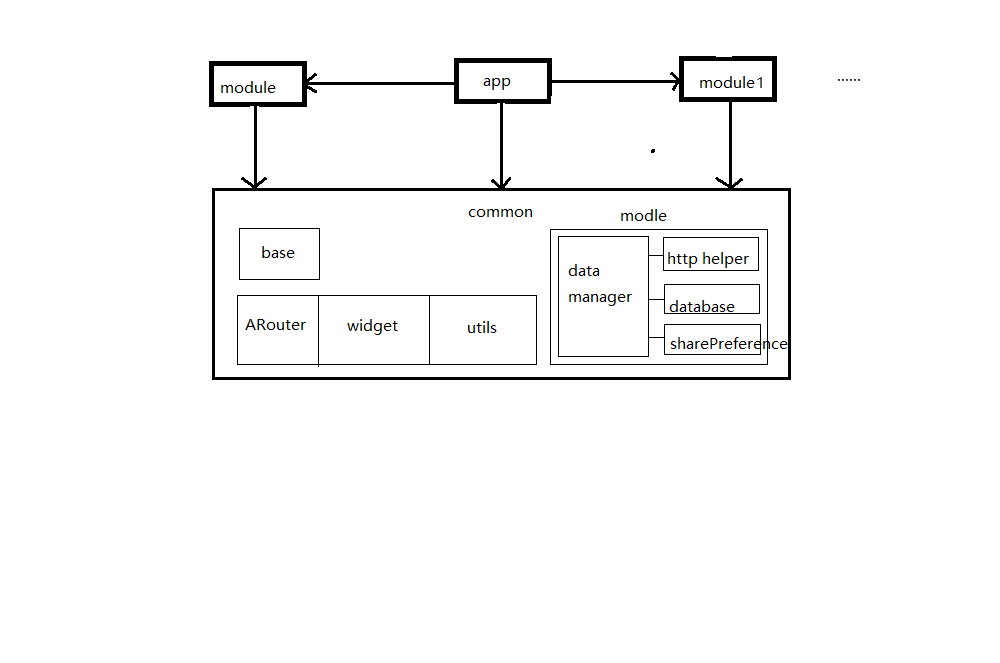
# 最新架构
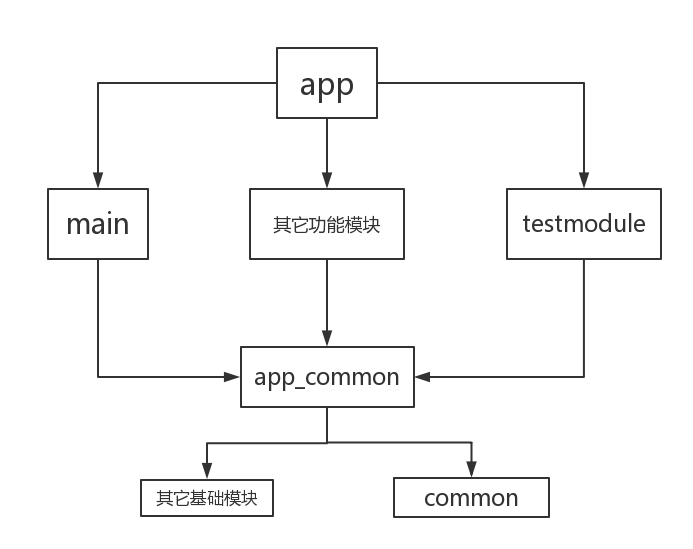
架构相关:app只作为壳存在,除了包含MyApplication、SplashActivity及跳往其它module的测试页面,不包含任何其它逻辑
功能模块之间跳转还是通过ARouter,模块间服务接口暴露于app_common中,使用服务的模块通过ARouter获取服务,模块之间完全解
耦;各模块中有xxxModule类,主要承担应用启动时的各模块初始化,也是通过ARouter获取调用;本次架构主要由ARouter承担大部分功能实现
再次跪谢!在项目build.gradle中配置需要参与编译的模块;具体使用见源码!
# License
```
Copyright 2017 aritraroy
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
```
| 0 |
wfh45678/radar | 实时风控引擎(Risk Engine),自定义规则引擎(Rule Script),完美支持中文,适用于反欺诈(Anti-fraud)应用场景,开箱即用!!!移动互联网时代的风险管理利器,你 Get 到了吗? | anti-fraud engine java radar real-time risk risk-analysis risk-control risk-engine risk-rule rule script springboot | # 风控引擎(Radar)
## 项目介绍
一款基于java语言,使用Springboot + Mongodb + Groovy + Es等框架搭建的轻量级实时风控引擎,适用于反欺诈应用场景,极简的配置,真正做到了开箱即用。
通过学习本项目能快速了解**风险的定义**,进而**量化风险** ,最后达到**集中管理风险**的目的。
A real-time risk analysis engine,which can update risk rule in real-time and make it effective immediately.
It applies to the anti-fraud application perfectly.
The project code called Radar, like the code, monitor the transaction at the back.
## 项目特点
* 实时风控,特殊场景可以做到100ms内响应
* 可视化规则编辑器,丰富的运算符、计算规则灵活
* 支持中文,易用性更强
* 自定义规则引擎,更加灵活,支持复杂多变的场景
* 插件化的设计,快速接入其它数据能力平台
* NoSQL,易扩展,高性能
* 配置简单,开箱即用!
## 相关站点
Gitee: https://gitee.com/freshday/radar
Github: https://github.com/wfh45678/radar // github 为镜像网站,贡献代码请提交到 gitee
官网: https://www.riskengine.cn
Wiki: https://gitee.com/freshday/radar/wikis/home
## 背景
伴随着移动互联网的高速发展,羊毛党快速崛起,从一平台到另一个平台,所过之处一地鸡毛,这还不是最可怕的,
随之而来的黑产令大部分互联网应用为之胆寒,通常新上线的APP的福利比较大,风控系统不完善,BUG 被发现的频率也比较高,
黑产利用BUG短时间给平台带来了巨大的损失,某多多的(100元测试优惠券,一夜损失上百万W)就是一例。
针对这一现象, 拥有一款实时的风控引擎是所有带有金融性质的APP 的当务之急,Radar应景而生。
Radar前身是笔者前公司的一个内部研究项目,由于众多原因项目商业化失败,考虑到项目本身的价值,弃之可惜,
现使用Springboot进行重构,删除了很多本地化功能,只保留风控引擎核心,更加通用,更加轻量,二次开发成本低,
开源出来,希望能给有风控需求的你们带来一些帮助。
## 项目初衷
我们知道企业做大后,会有很多产品线,而几乎每一个产品都需要做风险控制,通常我们都是把风险控制的逻辑写在相应的业务功能代码里,
大量重复的风控逻辑代码耦合在我们的业务逻辑里面,随着时间的累积,代码会变得异常复杂,会给后期的维护造成巨大的人力成本和风险。
所以风险的集中化管理势在必行,只有通过一个统一的管理平台,使用规则引擎,采用可视化配置的形式,
平台化管理不同产品的风控策略才是一种更好的方式, 而这正是Radar的初衷。
## 项目架构
前后端分离架构
后端技术框架: SpringBoot + Mybatis + tkMapper + Mysql + MongoDB + Redis + Groovy + ES + Swagger
前端技术框架: React(SPA)
### 架构图

## 技术选型
* Springboot:笔者是java 出生, 选择 Springboot 理所当然,方便自己, 也方便其他Java使用者进行扩展。
* Mybatis + tkMapper: 持久层框架, tkMapper 提供mapper 通用模板功能,减少重复代码的生成。
* Mysql : 本项目中关系数据库,主要用于存放 风险模型的元信息。
* MongoDB: 用于存放事件JSON, 提供基本统计学计算(例如:max, min, sum, avg,),
复杂的统计学概念(sd,variance, etc...)在内存中计算。
* ES: 提供数据查询和规则命中报表服务。
* Redis: 提供缓存支持,Engine 利用发布订阅特性监听管理端相关配置的更新
* Groovy: 规则引擎,风控规则最后都生成 groovy 脚本, 实时编辑,动态生成,即时生效。
* Swagger: Rest API 管理
---
## [使用手册](https://gitee.com/freshday/radar/wikis/manual)
使用手册里面有大量的图片,为了方便国内用户使用,故推荐码云的wiki 链接,
https://gitee.com/freshday/radar/wikis/manual
## 演示入口
通过管理端能够快速了解系统是怎么从风险的定义到风险的量化再到风险的集中管理的整个工作流程。
为了更好的体验,请花一分钟观看 [使用手册](https://gitee.com/freshday/radar/wikis/manual?sort_id=1637446)
[Demo URL:](https://www.riskengine.cn) https://www.riskengine.cn
建议大家自行注册用户,避免使用同样的测试账号受干扰.
## 相关文档
[WIKI:](https://gitee.com/freshday/radar/wikis/home?sort_id=1637444) https://gitee.com/freshday/radar/wikis/home?sort_id=1637444
## 致谢
感恩 XWF 团队,感谢参入的每一位小伙伴,后续征得同意后会一一列出名字。
千面怪, 烈日下的从容, DerekDingLu, king, sanying2012, 紫泉夜, 玄梦
成书平, 徐帅,郭锐, 王成,马兆永...
## 赞助商
* 中和农信项目管理有限公司
* 二十六度数字科技(广州)有限公司
感谢赞助商大大们对本项目的认可和支持。
## Contact to
如果喜欢本项目,Star支持一下, 让更多人了解本项目,谢谢!
## 特别说明
前端源码仅对企业级用户开放,需付费购买。
未经授权,禁止使用本项目源码申请软著和专利,保留追究法律责任的权力!
Copyright © 2019-2022 WangFeiHu
| 0 |
zhanglei-workspace/shopping-management-system | 该项目为多个小项目的集合(持续更新中...)。内容类似淘宝、京东等网购管理系统以及图书管理、超市管理等系统。目的在于便于Java初级爱好者在学习完某一部分Java知识后有一个合适的项目锻炼、运用所学知识,完善知识体系。适用人群:Java基础到入门的爱好者。 | java maven mybatis spring spring-mvc | README
===========================
前言:该项目是多个项目的合集,每个文件夹下存在一个Java项目。目的在于为不同知识层次的Java学习者提供一个项目实战晋级平台,便于Java爱好者有步伐频率的学习Java这门庞大的艺术体系。项目难度: `0-? --> 浅-深`。<br/>
☆☆☆<b>此项目代码仅可用于个人自我学习,谢绝任何组织或个人用于学生毕业设计、商业性传播等一切功利性行为。</b><br/>
☆tips: communication group number is 151162474 by qq.
****
Author: lyons(zhanglei) E-mail:zhangleiworkspace@gmail.com
<a name="index"/>目录
* [0:Java基础项目](#project0)
* 具备知识
- [x] sql
- [x] JDBC
- [x] Java基础
* [1:Java菜鸟项目](#project1)
* 具备知识
- [x] sql
- [x] JDBC
- [x] Java基础
- [x] HTML/CSS
- [x] JSP/Servlet
* [2:Java入门项目](#project2)
* 具备知识
- [x] sql
- [x] Java基础
- [x] HTML/CSS
- [x] JSP/Servlet
- [x] MyBatis
- [x] JavaScript
* [3:Java狙击项目](#project3)
* 具备知识
- [x] Maven
- [x] Shiro
- [x] Mybatis
- [x] Spring
- [x] SpringMVC
- [x] jQuery
* 包括但不限于上述所列知识...
<br><br>
<a name="project0"/>___0 Java基础项目___
#### Java控制台显示界面。
###
Java基础项目(商超购物管理系统)具有商品管理、前台收银、商品库存等功能,本项目使用java作为开发语言,
`原生JDBC`连接Oracle数据库存储数据,可以有效地锻炼和加强开发者运用Java、oracle数据库及基本sql编程开发的能力。
本项目适用于有Java、基本sql基础的开发者进行实战训练。(基础不错的同学可以直接学习 1# Java菜鸟项目 )
另:后续项目均以其上级项目为雏形进行新知识的增添进行代码重构来达到新知识应用学习的目的。
<br><br>
<a name="project1"/>___1 Java菜鸟项目___
#### JSP显示页面,模拟购物网站。
###
时间断断续续...
目前推送完成 注册、登陆、浏览商品、购物车、查询商品、退出 等模块。
此项目雏形完成,后续改进细节。相对于 《0# Java基础项目》 本项目知识量较大,慢慢消化。
后续:此项目不再进行更新维护,学习者学完此项目即可进入 《2:Java入门项目》
通过这两个项目的锻炼,可以尝试找份实习啦。
<br><br>
<a name="project2"/>___2 Java入门项目___
#### 改为mybatis连接数据库。
###
此项目代码根据 Java菜鸟项目 重构而来。
知识量逐步增大,本项目主要学习面向接口结合配置文件编程。
与以上两个项目显然的不同是,代码相对规范,接近企业级的编程思想。
利用周末空闲,逐渐推送中。。。
后续:该项目重构完成,仅再改进细枝末节。
小建议:以 表(Table) 为学习路径;Goods->Order->User.
预:《3:Java狙击项目》继续以重构的方式学习新知识。
需要学习者了解 maven、Spring MVC 等相关知识
因已使用mybatis故Hibernate不再在此项目中涉及,
两个者思想类似、职能相同,喜欢的同学可以自己研究重构。
<br><br>
<a name="project3"/>___3 Java狙击项目___
#### 飞鸟速购
###
该项目独立于上述项目存在。
此项目涉猎的知识更为广泛。
时间仍然很难连续起来,间断性推送中。。。
开发工具:Eclipse + Oracle
开发框架:shiro+spring+springMVc+mybatis
另:jdk 1.8
| 0 |
jodconverter/jodconverter | JODConverter automates document conversions using LibreOffice or Apache OpenOffice. | converter java libreoffice openoffice | # <img src="https://github.com/jodconverter/jodconverter/wiki/images/jodconverter_w200.png"> <sup> LibreOffice</sup> / <sub>Apache OpenOffice</sub>
[](https://cirrus-ci.com/github/jodconverter/jodconverter)
[](https://coveralls.io/github/jodconverter/jodconverter?branch=master)
[](https://www.codacy.com/gh/jodconverter/jodconverter/dashboard?utm_source=github.com&utm_medium=referral&utm_content=jodconverter/jodconverter&utm_campaign=Badge_Grade)
[](https://opensource.org/licenses/Apache-2.0)
[](https://maven-badges.herokuapp.com/maven-central/org.jodconverter/jodconverter-local)
[](http://javadoc.io/doc/org.jodconverter/jodconverter-local)
[](https://gitter.im/jodconverter/Lobby?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
[](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=XUYFM5NLLK628)
### What you want to know...
- **Documentation**: The JODConverter documentation (work in progress) can be found [here](https://github.com/jodconverter/jodconverter/wiki).
- **Examples**: A dedicated repository with sample projects can be found [here](https://github.com/jodconverter/jodconverter-samples).
- **Dependencies**:
* [jodconverter-local](https://maven-badges.herokuapp.com/maven-central/org.jodconverter/jodconverter-local) module dependencies.
* [jodconverter-remote](https://maven-badges.herokuapp.com/maven-central/org.jodconverter/jodconverter-remote) module dependencies.
* [jodconverter-spring](https://maven-badges.herokuapp.com/maven-central/org.jodconverter/jodconverter-spring) module dependencies.
* [jodconverter-spring-boot-starter](https://maven-badges.herokuapp.com/maven-central/org.jodconverter/jodconverter-spring-boot-starter) module dependencies.
- **Tests**: JODConverter is supposed to work just fine on recent versions of Windows, MacOS and Unix/Linux. Any confirmation would be welcome, so we could build a list of official supported OS distributions.
### Usage for local conversions
Build default, JODConverter is built using the OpenOffice libraries. See [here](https://github.com/jodconverter/jodconverter/issues/113) to know why. But you can now decide whether you want to use JODConverter with the LibreOffice libraries or the OpenOffice libraries.
#### With LibreOffice libraries:
#### Gradle:
```Shell
implementation 'org.jodconverter:jodconverter-local-lo:4.4.7'
```
#### Maven:
```Shell
<dependency>
<groupId>org.jodconverter</groupId>
<artifactId>jodconverter-local-lo</artifactId>
<version>4.4.7</version>
</dependency>
```
#### With OpenOffice libraries:
#### Gradle:
```Shell
implementation 'org.jodconverter:jodconverter-local:4.4.7'
```
or
```Shell
implementation 'org.jodconverter:jodconverter-local-oo:4.4.7'
```
#### Maven:
```Shell
<dependency>
<groupId>org.jodconverter</groupId>
<artifactId>jodconverter-local</artifactId>
<version>4.4.7</version>
</dependency>
```
or
```Shell
<dependency>
<groupId>org.jodconverter</groupId>
<artifactId>jodconverter-local-oo</artifactId>
<version>4.4.7</version>
</dependency>
```
### Building the Project
```Shell
gradlew clean build -x test
```
### Building Cli Executable
```Shell
gradlew clean build -x test distZip
```
#### Support <sup><sup>:speech_balloon:</sup></sup>
JODConverter Gitter Community [](https://gitter.im/jodconverter/Lobby?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge), growing [FAQ](https://github.com/jodconverter/jodconverter/wiki/FAQ).
### How to contribute
1. Check for [open issues](https://github.com/jodconverter/jodconverter/issues), or open a new issue to start a discussion around a feature idea or a bug.
2. If you feel uncomfortable or uncertain about an issue or your changes, feel free to contact me on Gitter using the link above.
3. [Fork this repository](https://help.github.com/articles/fork-a-repo/) on GitHub to start making your changes.
4. Write a test showing that the bug was fixed or that the feature works as expected.
5. Note that the repository follows the [Google Java style](https://google.github.io/styleguide/javaguide.html). You can format your code to this format by typing gradlew spotlessApply on the subproject you work on (e.g, `gradlew :jodconverter-local:spotlessApply`), by using the [Eclipse plugin](https://github.com/google/google-java-format#eclipse), or by using the [Intellij plugin](https://github.com/google/google-java-format#intellij).
6. [Create a pull request](https://help.github.com/articles/creating-a-pull-request/), and wait until it gets merged and published.
## Credits...
Here are my favorite/inspiration forks/projects:
- [documents4j project](https://github.com/documents4j/documents4j): Nice choice if you want 100% perfect conversion using MS Office. But work only on Windows out of the box (Local implementation) and not totally free (since MS Office is not free). The new "job" package is strongly inspired by this project.
## Original JODConverter
JODConverter (Java OpenDocument Converter) automates document conversions using LibreOffice or OpenOffice.org.
The previous home for this project is at [Google Code](http://code.google.com/p/jodconverter/),
including some [wiki pages](https://code.google.com/archive/p/jodconverter/wikis).
## Donations
If this project helps you, please consider a cup of :coffee:. Thanks!! :heart:
[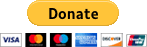](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=XUYFM5NLLK628)
| 0 |
bbottema/simple-java-mail | Simple API, Complex Emails (Jakarta Mail smtp wrapper) | batch-processing builder-api cli-support configurable conversion dkim dmarc email high-performance jakarta-mail java java-mail javamail oauth2 outlook proxy smime smtp smtp-client spring-support | [](modules/simple-java-mail/LICENSE-2.0.txt)
[](https://search.maven.org/#search%7Cgav%7C1%7Cg%3A%22org.simplejavamail%22%20AND%20v%3A%228.8.3%22)
[](https://www.javadoc.io/doc/org.simplejavamail/maven-master-project)
[](https://app.codacy.com/gh/bbottema/simple-java-mail)

# Simple Java Mail #
Simple Java Mail is the simplest to use lightweight mailing library for Java, while being able to send complex emails including **[Batch processing and server clusters](https://www.simplejavamail.org/configuration.html#section-batch-and-clustering)**, **[CLI support](https://www.simplejavamail.org/cli.html#navigation)**, **[authenticated socks proxy](https://www.simplejavamail.org/features.html#section-proxy)**(!), **[attachments](https://www.simplejavamail.org/features.html#section-attachments)**, **[embedded images](https://www.simplejavamail.org/features.html#section-embedding)**, **[custom headers and properties](https://www.simplejavamail.org/features.html#section-custom-headers)**, **[robust address validation](https://www.simplejavamail.org/features.html#section-email-validation)**, **[build pattern](https://www.simplejavamail.org/features.html#section-builder-api)** and even **[DKIM signing](https://www.simplejavamail.org/features.html#section-dkim)**, **[S/MIME support](https://www.simplejavamail.org/features.html#section-sending-smime)** and **[external configuration files](https://www.simplejavamail.org/configuration.html#section-config-properties)**, **[Spring support](https://www.simplejavamail.org/configuration.html#section-spring-support)** and **[Email conversion](https://www.simplejavamail.org/features.html#section-converting)** tools (including support for Outlook).
Just send your emails without dealing with [RFCs](https://www.simplejavamail.org/rfc-compliant.html#navigation).
The Simple Java Mail library is a thin layer on top of [Angus Mail](https://eclipse-ee4j.github.io/angus-mail/) (previously [Jakarta Mail](https://jakartaee.github.io/mail-api/README-JakartaMail)) that allows users to define emails on a high abstraction level without having to deal with mumbo jumbo such as 'multipart' and 'mimemessage'.
### [simplejavamail.org](https://www.simplejavamail.org) ###
Simple Java Mail is available in [Maven Central](https://search.maven.org/search?q=g:org.simplejavamail):
```xml
<dependency>
<groupId>org.simplejavamail</groupId>
<artifactId>simple-java-mail</artifactId>
<version>8.8.3</version>
</dependency>
```
Read about additional modules you can add here: [simplejavamail.org/modules](https://www.simplejavamail.org/modules.html).
### Latest Progress ###
v8.8.0 - [v8.8.3](https://repo1.maven.org/maven2/org/simplejavamail/simple-java-mail/8.8.3/)
- v8.8.3 (13-April-2024): [#502](https://github.com/bbottema/simple-java-mail/issues/502): [Bug] Message headers not treated with case insensitivity as per RFC, causing deviating headers to slip through the filters
- v8.8.2 (05-April-2024): [#495](https://github.com/bbottema/simple-java-mail/issues/495): Add config support for 'verifyingServerIdentity' with SMTP, also: since Angus 1.1.0 (8.6.0) server identity checks are on by default and can be countered by mailerBuilder.verifyingServerIdentity(false)
- v8.8.2 (05-April-2024): [#501](https://github.com/bbottema/simple-java-mail/issues/501): [dependency] Update outlook-message-parser dependency, which has improved support for X500 addresses
- v8.8.2 (05-April-2024): [#499 (fix)](https://github.com/bbottema/simple-java-mail/issues/499): [maintenance] Added missing finer-grained DKIM Spring Boot properties
- v8.8.1 (04-April-2024): [#500](https://github.com/bbottema/simple-java-mail/issues/500): [bug] Fix parsing addresses from headers in EML files, like a Disposition-Notification-To with umlaut
- v8.8.0 (22-March-2024): [#499](https://github.com/bbottema/simple-java-mail/issues/499): [Enhancement] Expose finer-grained DKIM configuration through the builder api and disable 'l-param' by default)
NOTE: release 8.8.0 changes the default for DKIM signing from 'l-param' true to false. If you rely on this feature, you need to enable it explicitly. Refer to the [DKIM documentation](https://www.simplejavamail.org/security.html#section-sending-dkim) for the update.
v8.7.0 - [v8.7.1](https://repo1.maven.org/maven2/org/simplejavamail/simple-java-mail/8.7.1/)
- v8.7.1 (20-March-2024): [#498](https://github.com/bbottema/simple-java-mail/issues/498): Make S/MIME algorithms configurable (signature algorithm for signing, key encapsulation and cipher algorithms for encryption)
- v8.7.1 (20-March-2024): [#497](https://github.com/bbottema/simple-java-mail/issues/497): Order of attachments is lost when converting a MimeMessage to an Email
- v8.7.0 (20-March-2024): don't use this version: versioning messed up
NOTE: this breaks the API for S/MIME related builder methods. Refer to the [S/MIME documentation](https://www.simplejavamail.org/security.html#section-sending-smime) for the new API.
v8.6.0 - [v8.6.3](https://repo1.maven.org/maven2/org/simplejavamail/simple-java-mail/8.6.3/)
- v8.6.3 (13-February-2024): [#491](https://github.com/bbottema/simple-java-mail/issues/491): [bug] Attachment body parts should separately parse Content-Disposition and ContentID, possible resulting in an downloadable attachment that is also embedded
- v8.6.2 (27-January-2024): [#493](https://github.com/bbottema/simple-java-mail/issues/493): [bug] don't require smime-module when adding collection of headers (also used when copying email)
- v8.6.1 (18-January-2024): [#487](https://github.com/bbottema/simple-java-mail/issues/487): Move header filtering from MimeMessageParser to EmailConverter, thereby enabling access to all parsed headers when using MimeMessageParser directly
- v8.6.1 (18-January-2024): [#489](https://github.com/bbottema/simple-java-mail/issues/489): Finished update to Angus Mail by updating activation dependency
- v8.6.0 (17-January-2024): [#489](https://github.com/bbottema/simple-java-mail/issues/489): Update to Angus Mail
NOTE: this release switches to Angus Mail which should be a transparent change, but if you encounter any issues, please report them.
One known issue is that Angus, since 1.1.0, performs server identity checks by default, which was previously disabled for SMTP. If you encounter issues with this, you can disable it with `mailerBuilder.verifyingServerIdentity(false)` and starting from 8.8.2, this also works with SMTP transport strategy (see [#495](https://github.com/bbottema/simple-java-mail/issues/495)).
v8.5.0 - [v8.5.1](https://repo1.maven.org/maven2/org/simplejavamail/simple-java-mail/8.5.1/)
- v8.5.1 (15-December-2023): [#486](https://github.com/bbottema/simple-java-mail/issues/486): [dependency] Handle Outlook's Non-Standard S/MIME Signed Messages
- v8.5.0 (13-December-2023): [#484](https://github.com/bbottema/simple-java-mail/issues/484): [bug] Addresses passed as string are not always interpreted correctly
[v8.4.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C8.4.0%7Cjar) (12-December-2023)
- [#483](https://github.com/bbottema/simple-java-mail/issues/483): Enhancement: add native support for overriding envelope-level receiver(s)
v8.3.0 - [v8.3.5](https://repo1.maven.org/maven2/org/simplejavamail/simple-java-mail/8.3.5/)
- v8.3.5 (10-December-2023): [#482](https://github.com/bbottema/simple-java-mail/issues/482) Bug: 'IllegalArgumentException: emailAddressList is required' when parsing mail with incorrect recipients from Outlook message
- v8.3.4 (08-December-2023): [#481](https://github.com/bbottema/simple-java-mail/issues/481) Enhancement: don't crash on invalid empty embedded images when parsing Outlook messages
- v8.3.3 (03-December-2023): [#477](https://github.com/bbottema/simple-java-mail/issues/477) Enhancement: Support Exchange proprietary addresses (X.500 DAP)
- v8.3.2 (26-November-2023): [#480](https://github.com/bbottema/simple-java-mail/issues/480) Bug: Multiple attachments with same name get the same Content-ID, causing them to refer to the same file content
- v8.3.1 (09-October-2023): [#440](https://github.com/bbottema/simple-java-mail/issues/440) Bug: names manually specified for embedded images are overridden and have extension added, breaking cid: references in HTML body
- v8.3.0 (09-October-2023): [#475](https://github.com/bbottema/simple-java-mail/issues/475) Enhancement: Add configuration metadata for Spring Boot application properties
[v8.2.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C8.2.0%7Cjar) (02-October-2023)
- [#473](https://github.com/bbottema/simple-java-mail/issues/473) Bugfix: Add missing support for multiple reply-to addresses
v8.1.0 - [v8.1.3](https://repo1.maven.org/maven2/org/simplejavamail/simple-java-mail/8.1.3/)
- v8.1.3 (14-July-2023): [#467](https://github.com/bbottema/simple-java-mail/issues/467) Security: Medium severity vulnerability is detected in org.bouncycastle transitive dependency
- v8.1.3 (14-July-2023): [#466](https://github.com/bbottema/simple-java-mail/issues/466) Maintenance: Let Mailer implement AutoCloseable, so it shuts down the connection pool automatically when disposed of by Spring
- v8.1.2 (23-June-2023): [#465](https://github.com/bbottema/simple-java-mail/issues/465) Regression bug #461: Simple Java Mail always requires DKIM/SMIME modules
- v8.1.1 (07-June-2023): [#461](https://github.com/bbottema/simple-java-mail/issues/461) Bugfix: Fixed MessageID not preserved when signing/encrypting with S/MIME and/or DKIM
- v8.1.0 (15-April-2023): [#458](https://github.com/bbottema/simple-java-mail/pull/458) Missing osgi headers (#288) and added support for Apache Karaf
- v8.1.0 (15-April-2023): [#288](https://github.com/bbottema/simple-java-mail/issues/288) Maintenance: missing OSGI package-exports from core-module
v8.0.0 - [v8.0.1](https://repo1.maven.org/maven2/org/simplejavamail/simple-java-mail/8.0.1/)
- v8.0.1 (30-April-2023): [#456](https://github.com/bbottema/simple-java-mail/issues/456): Enhancement: make Content-Transfer encoder detection more lenient, supporting more values from the wild
- v8.0.0 (08-March-2023): [#451](https://github.com/bbottema/simple-java-mail/issues/451): Feature: Make defaults and overrides a first class feature
- v8.0.0 (08-March-2023): [#452](https://github.com/bbottema/simple-java-mail/issues/452): Enhancement: with ".disableAllClientValidation(true)", also ignore errors from the completeness check
- v8.0.0 (08-March-2023): [#450](https://github.com/bbottema/simple-java-mail/issues/450): Bug: when using dispositionNotificationTo or returnReceiptTo mode, when the corresponding emails are not filled, it fails even though it should fall back to replyTo or From
- v8.0.0 (08-March-2023): [#449](https://github.com/bbottema/simple-java-mail/issues/449): Bug: IllegalArgumentException on parsing empty header name and value (when parsing Outlook message)
- v8.0.0 (08-March-2023): [#448](https://github.com/bbottema/simple-java-mail/issues/448): Bug: withEmailDefaults and withEmailOverrides does not work with CustomMailer
- v8.0.0 (08-March-2023): [#447](https://github.com/bbottema/simple-java-mail/issues/447): Enhancement: allow defaults/overrides to ignore individual fields (turn off for specific properties)
- v8.0.0 (08-March-2023): [#446](https://github.com/bbottema/simple-java-mail/issues/446): Enhancement: add missing defaults properties for DKIM
**This release changes how Email instances are built, or more specifically, when defaults and overrides are applied.
There are now overloaded build methods that provide similar behaviour as previous versions.**
v7.9.0 - [v7.9.1](https://repo1.maven.org/maven2/org/simplejavamail/simple-java-mail/7.9.1/)
- v7.9.1 (22-February-2023): [#444](https://github.com/bbottema/simple-java-mail/issues/444) Bugfix: encoded delimited recipients in EML not parsed properly
- v7.9.0 (21-February-2023): [#344](https://github.com/bbottema/simple-java-mail/issues/344) Enhancement: make DKIM signing more flexible by allowing header exclusions in DKIM signature
v7.8.0 - [v7.8.3](https://repo1.maven.org/maven2/org/simplejavamail/simple-java-mail/7.8.3/)
- v7.8.3 (21-February-2023): [#293](https://github.com/bbottema/simple-java-mail/issues/293) Bugfix: Decoding missing in a few placed when parsing MimeMessage or sending an Email
- v7.8.2 (09-February-2023): [#442](https://github.com/bbottema/simple-java-mail/issues/442) Enhancement: Simple Java Mail should throw an exception when trying to utilize S/MIME with smime-module on the classpath
- v7.8.1 (01-February-2023): [#438](https://github.com/bbottema/simple-java-mail/issues/438) Bug: properly Fail-Fast in case of Transport claim timeout in the batch-module, rather than running into NPE further down the line
- v7.8.0 (24-January-2023): [#436](https://github.com/bbottema/simple-java-mail/issues/436) Enhancement: add mailerBuilder.withTransportModeLoggingOnly() as mailer API entry point
- v7.8.0 (24-January-2023): [#435](https://github.com/bbottema/simple-java-mail/issues/435) Enhancement: SMTP server config should be optional in case a CustomMailer is used
- v7.8.0 (24-January-2023): [#427](https://github.com/bbottema/simple-java-mail/issues/427) Feature: set a maximum email size on Mailer level which throws EmailToBig exception when exceeded
v7.7.0 - [v7.7.1](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C7.7.1%7Cjar)
- v7.7.1 (18-January-2023): [#434](https://github.com/bbottema/simple-java-mail/issues/434) Regression bug in #430: Email parameter missing in CustomMailer interface
- v7.7.0 (17-January-2023): [#430](https://github.com/bbottema/simple-java-mail/issues/430) Enhancement: auto-reconnect (if needed) when reclaiming a Transport connection from the SMTP connection
- v7.7.0 (17-January-2023): [#383](https://github.com/bbottema/simple-java-mail/issues/383) Feature: be able to set defaults and overrides on the Mailer level, rather than email or global level
7.7.0 moves the conversion of Email to MimeMessage to after a Transport instance has been selected (in case of a cluster of SMTP servers),
so we can apply defaults/overrides on the Mailer level, meaning you can configure 'global' values for individual SMTP servers
(like a fixed FROM per server).
[v7.6.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C7.6.0%7Cjar) (05-January-2023)
- [#421](https://github.com/bbottema/simple-java-mail/issues/421) Enhancement: Add support for OAUTH2 authentication
v7.5.0 - [v7.5.2](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C7.5.2%7Cjar)
- v7.5.2 (25-December-2022): [#429](https://github.com/bbottema/simple-java-mail/issues/429) Bug: wrong username property used when password authentication is not needed
- v7.5.1 (12-December-2022): [#416](https://github.com/bbottema/simple-java-mail/issues/416) Bug: Support encoder names regardless of their case ("base64" is the same as "BASE64")
- v7.5.1 (12-December-2022): [#424](https://github.com/bbottema/simple-java-mail/issues/424) Maintenance: bump JMail dependency from 1.2.1 to 1.4.1
- v7.5.0 (28-July-2022): [#411](https://github.com/bbottema/simple-java-mail/issues/411) Enhancement: expose validation sub steps in the MailerHelper class for the completeness check, CRLF inject scans and address validations
- v7.5.0 (28-July-2022): [#410](https://github.com/bbottema/simple-java-mail/issues/410) Bug: CRLF injection scan missing for dispositionNotificationTo and returnReceiptTo
- v7.5.0 (28-July-2022): [#390](https://github.com/bbottema/simple-java-mail/issues/390) Enhancement: make client sided validation optional, turning off address validation and CRLF injection detection
[v7.5.1](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C7.5.1%7Cjar) (12-December-2022)
- [#416](https://github.com/bbottema/simple-java-mail/issues/416) Bug: Support encoder names regardless of their case ("base64" is the same as "BASE64")
- [#424](https://github.com/bbottema/simple-java-mail/issues/424) Maintenance: bump JMail dependency from 1.2.1 to 1.4.1
[v7.5.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C7.5.0%7Cjar) (28-July-2022)
- [#411](https://github.com/bbottema/simple-java-mail/issues/411) Enhancement: expose validation sub steps in the MailerHelper class for the completeness check, CRLF inject scans and address validations
- [#410](https://github.com/bbottema/simple-java-mail/issues/410) Bug: CRLF injection scan missing for dispositionNotificationTo and returnReceiptTo
- [#390](https://github.com/bbottema/simple-java-mail/issues/390) Enhancement: make client sided validation optional, turning off address validation and CRLF injection detection
[v7.4.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C7.4.0%7Cjar) (19-July-2022)
- [#407](https://github.com/bbottema/simple-java-mail/issues/407) Enhancement: Process all Outlook message headers, either copying the as-is or translating them to respective Simple Java Mail API calls
- [#404](https://github.com/bbottema/simple-java-mail/issues/404) Minor bugfix: the new attachment's contentDescription was missing in Email.toString()
[v7.3.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C7.3.0%7Cjar) (15-July-2022)
- [#405](https://github.com/bbottema/simple-java-mail/issues/405) Feature: Expand email builder API to support forced content Content-Transfer-Encoding for attachments, like quoted-printable, base64, 7BIT and others
- [#404](https://github.com/bbottema/simple-java-mail/issues/404) Feature: Expand email builder API to support Content-Description on attachments
v7.2.0 - [v7.2.1](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C7.2.1%7Cjar)
- v7.2.1 (13-July-2022): [#396](https://github.com/bbottema/simple-java-mail/issues/396) Enhancement: make Outlook support tolerant of invalid/empty nested Outlook message attachments
- v7.2.0 (13-July-2022): [#399](https://github.com/bbottema/simple-java-mail/issues/399) Feature: Expand email builder API to support [selective content encoding](https://www.simplejavamail.org/features.html#section-content-transfer-encoding), like quoted-printable, base64, 7BIT and others
v7.1.0 - [v7.1.3](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C7.1.3%7Cjar)
- v7.1.3 (12-July-2022): [#403](https://github.com/bbottema/simple-java-mail/issues/403) Security: Bump zip4j (only used during testing)
- v7.1.2 (12-July-2022): [#401](https://github.com/bbottema/simple-java-mail/issues/401) Enhancement: Add HEIC and WEBP support when dynamically resolving embedded images from classpath
- v7.1.2 (12-July-2022): [#402](https://github.com/bbottema/simple-java-mail/issues/402) Security: Update Log4j to 2.17.1
- v7.1.2 (12-July-2022): [#393](https://github.com/bbottema/simple-java-mail/issues/393) Security: Update Apache POI and POI Scratchpad
- v7.1.1 (27-March-2022): [#387](https://github.com/bbottema/simple-java-mail/issues/387) Bug: memory leak in SMPT connection pool when 3rd party deallocation failed with exception
- v7.1.0 (25-January-2022): [#379](https://github.com/bbottema/simple-java-mail/issues/379) Maintenance: Adjust dependencies and make Java 9+ friendly
v7.0.1 - [v7.0.2](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C7.0.2%7Cjar)
- v7.0.2 (25-January-2022): [#329](https://github.com/bbottema/simple-java-mail/issues/329) Enhancement: Exceptions cause error-level logging in addition to rethrowing the exception, but should just include the message in a custom exception
- v7.0.2 (25-January-2022): [#378](https://github.com/bbottema/simple-java-mail/issues/378) Bug: package org.simplejavamail.internal.modules causes split package problem in Java9+
- v7.0.1 (22-January-2022): [#375](https://github.com/bbottema/simple-java-mail/issues/375) Bug: [batch-module](https://www.simplejavamail.org/features.html#section-sending-asynchronously) gives error when there is a [custom mailer](https://www.simplejavamail.org/features.html#section-custom-mailer)
[v7.0.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C7.0.0%7Cjar) (2-January-2022)
It has been two years since the last major release, but 7.0.0 finally here!
#### What's new ####
Major features:
- [#322](https://github.com/bbottema/simple-java-mail/issues/322) Simple Java Mail migrated to Java 8 finally (see notes below)
- [#295](https://github.com/bbottema/simple-java-mail/issues/295) And also switched to JavaMail's successor Jakarta Mail 2.0.1 (see notes below)
- [#323](https://github.com/bbottema/simple-java-mail/issues/323) Solved the great CLI performance problem (now executes near instantly)
- [#319](https://github.com/bbottema/simple-java-mail/issues/319) Replaced the underlying regex-based email-address validation library with the lexer based [JMail](https://github.com/RohanNagar/jmail), which is faster, [correcter](https://www.rohannagar.com/jmail/), documented better and is more up-to-date with RFC's
- [#367](https://github.com/bbottema/simple-java-mail/issues/367) The sendMail/testConnection methods now have proper support for `CompletableFuture`
Bugfixes:
- [#352](https://github.com/bbottema/simple-java-mail/issues/352) Bug: names regex groups are not supported in Android JVM
- [#326](https://github.com/bbottema/simple-java-mail/issues/326) Bug: NullPointer when parsing Outlook Message with nested empty Outlook message
- [#330](https://github.com/bbottema/simple-java-mail/issues/330) Bug: cli expected --mailer arguments duplicated 3 times
- [#324](https://github.com/bbottema/simple-java-mail/issues/324) Bug: Add back missing log4j2 for CLI library
Maintenance:
- [#368](https://github.com/bbottema/simple-java-mail/issues/368) Resolve log4j (Java8) [vulnerability](https://logging.apache.org/log4j/2.x/security.html) in Simple Java Mail's CLI module
- [#330](https://github.com/bbottema/simple-java-mail/issues/330) Improved feedback from failing CLI commands
- [#327](https://github.com/bbottema/simple-java-mail/issues/327) Implement toString() for Mailer instances for debugging purposes
#### About the migration ####
Updating to Java8/Jakarta 2.0.1 posed a challenge as the 3rd party S/MIME library [java-utils-mail-smime](https://github.com/markenwerk/java-utils-mail-smime) has been abandoned/archived while developing Simple Java Mail. Furthermore, it was still under LGPL3 license while everything else is ApacheV2.
Thankfully, I obtained permissions from the maintainers -as well as original developers from decades ago on SourceForge- to take both java-utils-mail-smime and java-utils-mail-dkim under my wings at Simple Java Mail and change the licensing model! You can now post issues and pull requests here:
- [simple-java-mail/java-utils-mail-smime](https://github.com/simple-java-mail/java-utils-mail-smime)
- [simple-java-mail/java-utils-mail-dkim](https://github.com/simple-java-mail/java-utils-mail-dkim)
v6.7.0 - [v6.7.6](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C6.7.6%7Cjar)
- v6.7.6 (22-January-2022): [#375](https://github.com/bbottema/simple-java-mail/issues/375) Bug: [batch-module](https://www.simplejavamail.org/features.html#section-sending-asynchronously) gives error when there is a [custom mailer](https://www.simplejavamail.org/features.html#section-custom-mailer)
- v6.7.5 (26-December-2021): [#338](https://github.com/bbottema/simple-java-mail/issues/338) Enhancement: Also return AsyncResponse from plain Mailer.sendEmail(singleArgument) as async can be configured through MailerBuilder now
- v6.7.4 (25-December-2021): [#331](https://github.com/bbottema/simple-java-mail/issues/331) Enhancement: Coalesce empty SMTP server arguments to null to support CLI better
- v6.7.3 (25-December-2021): [#335](https://github.com/bbottema/simple-java-mail/issues/335) Bugfix: Precondition nonNull check also checks nonEmpty and breaks on clearEmailAddressCriteria
- v6.7.2 (25-December-2021): [#318](https://github.com/bbottema/simple-java-mail/issues/318) Maintenance: Allow zero data attachments so Outlook message conversions don't crash and burn
- v6.7.1 (25-December-2021): [#346](https://github.com/bbottema/simple-java-mail/issues/346) Bugfix: Add option to parse MimeMessage without fetching attachment data from server - Properly return named datasource without fetching all the data if unwanted
- v6.7.0 (25-December-2021): [#356](https://github.com/bbottema/simple-java-mail/issues/356) Enhancement: Improve for support for Android < 8.0 (Fix NoClassDefFoundError)
- v6.7.0 (25-December-2021): [#351](https://github.com/bbottema/simple-java-mail/issues/351) Bugfix: emlToEmail() and mimeMessageToEmail() break on mesages with duplicate names and legacy empty nested messages
- v6.7.0 (25-December-2021): [#347](https://github.com/bbottema/simple-java-mail/issues/347) Maintenance: Reduce log-spam and prevent exception on every module availability check
- v6.7.0 (25-December-2021): [#346](https://github.com/bbottema/simple-java-mail/issues/346) Feature: Add option to parse MimeMessage [without fetching attachment data](https://www.simplejavamail.org/features.html#section-converting) from server
v6.6.0 - [v6.6.2](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C6.6.2%7Cjar)
- v6.6.2 (23-December-2021): [#365](https://github.com/bbottema/simple-java-mail/issues/365) Security: Resolve log4j vulnerability in Simple Java Mail
- v6.6.1 (12-June-2021): [#321](https://github.com/bbottema/simple-java-mail/issues/321) Enhancement: Ignore malformed recipient addresses and continue parsing email data
- v6.6.0 (1-June-2021): [#320](https://github.com/bbottema/simple-java-mail/issues/320) Enhancement: Added [default S/MIME signing](https://www.simplejavamail.org/features.html#section-sending-smime) to Mailer level and fixed crippling performance bug
v6.5.0 - [v6.5.4](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C6.5.4%7Cjar)
- v6.5.4 (22-May-2021): [#315](https://github.com/bbottema/simple-java-mail/issues/315) Enhancement: Nested attachments of Outlook message attachments are now preserved as standard EML MimeMessage attachments
**NOTE:** This _removes_ the Kryo dependency
- v6.5.3 (4-May-2021): [#314](https://github.com/bbottema/simple-java-mail/issues/314) Bugfix: Nested attachments of Outlook message attachments are now preserved, by utilizing Kryo
**NOTE:** This add Kryo as extra dependency to the outlook-module (and is subsequently removed in 6.5.4)
- v6.5.2 (15-April-2021): [#311](https://github.com/bbottema/simple-java-mail/issues/311) Bugfix: text/calendar as string -> ClassCastException (if calendar type is not Inputstream)
- v6.5.1 (10-April-2021): [#307](https://github.com/bbottema/simple-java-mail/issues/307) / [#310](https://github.com/bbottema/simple-java-mail/issues/310) Bugfix: embedded image resource name got mangled
- v6.5.0 (16-February-2021): [#298](https://github.com/bbottema/simple-java-mail/issues/298) Enhancement: Nested Outlook messages aren't discarded anymore, but parsed to serialized Email objects
- v6.5.0 (16-February-2021): [#292](https://github.com/bbottema/simple-java-mail/issues/292) Bugfix: NullPointerException in SmimeUtilFixed when protocol is missing (which is valid)
- v6.5.0 (16-February-2021): [#289](https://github.com/bbottema/simple-java-mail/issues/289) Bugfix: Support multiple headers with same key
This release breaks all GET/SET api regarding headers used as map (Map<String, T> -> Map<String, Collection<T>>)
This release might break api in the rare case you relied on the attachment list and you have nested Outlook .msg attachments (previously omitted in the results)
v6.4.0 - [v6.4.5](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C6.4.5%7Cjar)
- v6.4.5 (13-Februari-2021): [#306](https://github.com/bbottema/simple-java-mail/issues/306) Maintenance: Update outlook-message-parser to 1.7.9
- v6.4.5 (13-Februari-2021): [#304](https://github.com/bbottema/simple-java-mail/issues/304) Regression bugfix: batch-module needed for sending mails async, basic version should work without
- v6.4.5 (13-Februari-2021): [#303](https://github.com/bbottema/simple-java-mail/issues/303) Bugfix: EML Attachments are modified/have the wrong size
- v6.4.4 (25-October-2020): [#294](https://github.com/bbottema/simple-java-mail/issues/294) Always invoke async success/exception handlers even if set after sending email (behaving more like promises/futures)
- v6.4.4 (25-October-2020): [#291](https://github.com/bbottema/simple-java-mail/issues/291) On Exception only log the email ID at error level and log the whole email at trace level
- v6.4.4 (25-October-2020): [#290](https://github.com/bbottema/simple-java-mail/issues/290) Only perform expensive logging logic if respective logging level is enabled
- v6.4.3 (6-August-2020): [#284](https://github.com/bbottema/simple-java-mail/issues/284) Improved support for Calendar attachments created by gMail
- v6.4.3 (6-August-2020): [#283](https://github.com/bbottema/simple-java-mail/issues/283) Bugfix: Fix support for reading Calendar attachments with quoted-printable transfer-type
- v6.4.2 (3-August-2020): [#281](https://github.com/bbottema/simple-java-mail/issues/281) Bugfix: Fix support for reading Calendar attachments
- v6.4.1 (26-July-2020): [#252](https://github.com/bbottema/simple-java-mail/issues/252) Bugfix: Added missing support for S/MIME enveloped signing
- v6.4.0 (19-July-2020): [#268](https://github.com/bbottema/simple-java-mail/issues/268) Immediately resolve InputStreams when building emails, don't reuse
* This primarily affects the builder api for S/MIME and DKIM signing / encryption.
v6.3.0 - [v6.3.2](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C6.3.2%7Cjar) (11-July-2020 - 12-July-2020)
- [#271](https://github.com/bbottema/simple-java-mail/issues/271) Bugfix: Attachment (file)names with special characters should not be encoded
- [#248](https://github.com/bbottema/simple-java-mail/issues/248) Bugfix: MimeMessageHelper: use complete filename as resource name
- [#279](https://github.com/bbottema/simple-java-mail/issues/279) Allow [extra Session properties](https://www.simplejavamail.org/features.html#section-custom-properties) configured through simplejavamail.properties
- [#277](https://github.com/bbottema/simple-java-mail/issues/277) Add API for using [custom SSLSocketFactory](http://localhost:3000/features.html#section-ssl-tls)
[v6.2.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C6.2.0%7Cjar) (9-July-2020)
This release adds the following major new feature:
- [#260](https://github.com/bbottema/simple-java-mail/issues/260) **Add support for [dynamic datasource resolution](https://www.simplejavamail.org/features.html#section-embedding)** (file/url/classpath) for embedded images in HTML body
[v6.1.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C6.1.0%7Cjar) (5-July-2020)
- [#264](https://github.com/bbottema/simple-java-mail/issues/264) Switch from AssertionError to IllegalStateException
- Bumped outlook-message-parser from 1.7.3 to 1.7.5
- bugfix for parsing chinese unsent Outlook messages
- bugfix Outlook attachments with special characters in the name
- Bumped email-rfc2822-validator from 2.1.3 to 2.2.0
- bugfix properly handle brackets in email addresses when allowed
- Bumped log4j-core from 2.6.1 to 2.13.2
v6.0.2 - [v6.0.5](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C6.0.5%7Cjar) (21-January-2020 - 13-June-2020)
- [#270](https://github.com/bbottema/simple-java-mail/issues/270) Bug: CLI module missing Jetbrains @Nullable annotation dependency needed in runtime
- [#262](https://github.com/bbottema/simple-java-mail/issues/262) Bug: Executor settings passed to the builder are ignored
- [#249](https://github.com/bbottema/simple-java-mail/issues/249) Bug: MimeMessageParser doesn't handle multiple attachments with the same name correctly
- [#245](https://github.com/bbottema/simple-java-mail/issues/245) Bug: JDK9+ Incorrect JPMS Automatic-Module-Name
- [#246](https://github.com/bbottema/simple-java-mail/issues/246) Bug: Sending async emails with and without the Batch module cause lingering threads
v6.0.0-rc1 - [v6.0.1](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C6.0.1%7Cjar) (18-December-2019 - 24-January-2020)
After almost two years of development the next major release 6.0.0 is finally here! And what a doozy it is, with the following major new features:
The core library is now even smaller compared to the 5.x.x series going from 183kb to 134kb!
- [CLI support!!](https://www.simplejavamail.org/cli.html#navigation),
- major performance improvement with [advanced batch processing](https://www.simplejavamail.org/configuration.html#section-batch-and-clustering) including support for mail server clusters.
- You can now replace the final sending of emails with [your own logic](https://www.simplejavamail.org/features.html#section-custom-mailer), using a 3rd party service of your choice.
- 6.0.0 also includes support for [S/MIME signed and encrypted emails](https://www.simplejavamail.org/features.html#section-smime)!
- All 3rd party dependencies have been made optional by splitting up Simple Java Mail into easy to use [modules](https://www.simplejavamail.org/modules.html#navigation).
- You can now monitor and [handle async processing](https://www.simplejavamail.org/features.html#section-handling-async-result) using Futures.
- MimeMessage results are now [structurally matched](https://www.simplejavamail.org/rfc-compliant.html#section-explore-multipart) to specific needs (only using alternative/mixed etc. when needed)
Here's the complete list of changes:
#### New features and enhancements ####
- [#183](https://github.com/bbottema/simple-java-mail/issues/183) To manage all the optional dependencies and related code, Simple Java Mail should be [split up into modules](https://www.simplejavamail.org/modules.html#navigation)
- [#156](https://github.com/bbottema/simple-java-mail/issues/156) Add [CLI support](https://www.simplejavamail.org/cli.html#navigation)
- [#214](https://github.com/bbottema/simple-java-mail/issues/214) Support more [advanced batch processing](https://www.simplejavamail.org/features.html#section-sending-asynchronously) use cases
- [#187](https://github.com/bbottema/simple-java-mail/issues/187) Simple Java Mail should have optional support for signed [S/MIME attachments](https://www.simplejavamail.org/modules.html#smime-module)
- [#121](https://github.com/bbottema/simple-java-mail/issues/121) Introduce interfaces for validation and sending, so these steps can be customized
- [#144](https://github.com/bbottema/simple-java-mail/issues/144) Simple Java Mail should [tailor the MimeMessage structure](https://www.simplejavamail.org/migration-notes-6.0.0.html#mimemessage-structure) to specific needs
- [#138](https://github.com/bbottema/simple-java-mail/issues/138) Add support for [Calendar events](https://www.simplejavamail.org/features.html#section-icalendar-vevent) (iCalendar vEvent)
- [#235](https://github.com/bbottema/simple-java-mail/issues/235) Be able to [fix the sent date](https://www.simplejavamail.org/features.html#section-custom-sentdate) for a new email
- [#232](https://github.com/bbottema/simple-java-mail/issues/232) Improve encoding of attachment file names
- [#222](https://github.com/bbottema/simple-java-mail/issues/222) Add config property support for trusting hosts and verifying server identity
- [#212](https://github.com/bbottema/simple-java-mail/issues/212) Authenticated proxy server started even if already running, raising exception
- [#207](https://github.com/bbottema/simple-java-mail/issues/207) Implement more comprehensive ThreadPoolExecutor and expose config options
- [#211](https://github.com/bbottema/simple-java-mail/issues/211) SpringSupport should expose the intermediate builder for customization
- [#193](https://github.com/bbottema/simple-java-mail/issues/193) Simple Java Mail should use default server ports when not provided by the user
#### Bugs solved ####
- [#242](https://github.com/bbottema/simple-java-mail/issues/242) Renamed log4j2.xml to log4j2_example.xml so it doesn't clash with project config
- [#241](https://github.com/bbottema/simple-java-mail/issues/241) EmailConverter.outlookMsgToEmail duplicates recipients
- [#239](https://github.com/bbottema/simple-java-mail/issues/239) List of Recipients not ordered as added (insertion order not maintained)
- [#236](https://github.com/bbottema/simple-java-mail/issues/236) Message ID should be mapped from Outlook messages as well
- [#210](https://github.com/bbottema/simple-java-mail/issues/210) Connection/session timeout properties not set when not sending in batch mode
- [#201](https://github.com/bbottema/simple-java-mail/issues/201) When parsing Outlook message, FROM address should default to a dummy address when missing
- [#200](https://github.com/bbottema/simple-java-mail/issues/200) When parsing Outlook message, attachment name doesn't fallback on filename if proper name is empty
- [#161](https://github.com/bbottema/simple-java-mail/issues/161) When reading (chinese) .msg files, HTML converted from RTF is completely garbled (encoding issue)
- [#159](https://github.com/bbottema/simple-java-mail/issues/159) Can not parse email with blank email address headers
- [#139](https://github.com/bbottema/simple-java-mail/issues/139) Multiple Bodyparts of same Content-Type not supported for text/html & text/plain within Multipart/mixed or Multipart/alternative
- [#151](https://github.com/bbottema/simple-java-mail/issues/151) Attachment's file extension overwritten by resource's invalid extension
#### Maintenance updates ####
- [#165](https://github.com/bbottema/simple-java-mail/issues/165) Move away from Findbugs (unofficial JSR-305) annotations
- [#164](https://github.com/bbottema/simple-java-mail/issues/164) The DKIM dependency has been updated to benefit from the newer Apache V2 license
- [#164](https://github.com/bbottema/simple-java-mail/issues/164) The DKIM dependency has been updated to benefit from the newer Apache V2 license
- [#184](https://github.com/bbottema/simple-java-mail/issues/184) Update JavaMail dependency to 1.6.2, adding support for UTF-8 charset
- [#186](https://github.com/bbottema/simple-java-mail/issues/186) Update JavaMail dependency to 1.6.2, adding support for authenticated HTTP web proxy
- [#146](https://github.com/bbottema/simple-java-mail/issues/146) Added OSGI manifest and switched to spotbugs
#### Included changes from outlook-message-parser ####
- v6.0.1, v1.7.3: [#27](https://github.com/bbottema/outlook-message-parser/issues/27) When from name/address are not available (unsent emails), these fields are filled with binary garbage
- v6.0.1, v1.7.2: [#26](https://github.com/bbottema/outlook-message-parser/issues/26) To email address is not handled properly when name is omitted
- v6.0.0, v1.7.1: [#25](https://github.com/bbottema/outlook-message-parser/issues/25) NPE on ClientSubmitTime when original message has not been sent yet
- v6.0.0, v1.7.1: [#23](https://github.com/bbottema/outlook-message-parser/issues/23) Bug: __nameid_ directory should not be parsed (and causing invalid HTML body)
- v6.0.0, v1.7.0: [#18](https://github.com/bbottema/outlook-message-parser/issues/18) Upgrade Apache POI 3.9 -> 4.x (but managed back for Simple Java Mail due to incompatibility with Java 7)
- v6.0.0, v1.6.0: [#21](https://github.com/bbottema/outlook-message-parser/issues/21) Multiple TO recipients are not handles properly
- v6.0.0, v1.5.0: [#20](https://github.com/bbottema/outlook-message-parser/issues/20) CC and BCC recipients are not parsed properly
- v6.0.0, v1.5.0: [#19](https://github.com/bbottema/outlook-message-parser/issues/19) Use real Outlook ContentId Attribute to resolve CID Attachments
- v6.0.0, v1.4.1: [#17](https://github.com/bbottema/outlook-message-parser/issues/17) Fixed encoding error for UTF-8's Windows legacy name (cp)65001
- v6.0.0, v1.4.0: [#9](https://github.com/bbottema/outlook-message-parser/issues/9) Replaced the RFC to HTML converter with a brand new RFC-compliant convert! (thanks to @fadeyev!)
- v6.0.0, v1.3.0: [#14](https://github.com/bbottema/outlook-message-parser/issues/14) Dependency problem with Java9+, missing Jakarta Activation Framework
- v6.0.0, v1.3.0: [#13](https://github.com/bbottema/outlook-message-parser/issues/13) HTML start tags with extra space not handled correctly
- v6.0.0, v1.3.0: [#11](https://github.com/bbottema/outlook-message-parser/issues/11) SimpleRTF2HTMLConverter inserts too many <br/> tags
- v6.0.0, v1.3.0: [#10](https://github.com/bbottema/outlook-message-parser/issues/10) Embedded images with DOS-like names are classified as attachments
- v6.0.0, v1.3.0: [#9](https://github.com/bbottema/outlook-message-parser/issues/9) SimpleRTF2HTMLConverter removes some valid tags during conversion
- v6.0.0, v1.2.1: Ignore non S/MIME related content types when extracting S/MIME metadata
- v6.0.0, v1.2.1: Added toString and equals methods to the S/MIME data classes
- v6.0.0, v1.1.21: Upgraded mediatype recognition based on file extension for incomplete attachments
- v6.0.0, v1.1.21: Added / improved support for public S/MIME meta data
- v6.0.0, v1.1.20: [#7](https://github.com/bbottema/outlook-message-parser/issues/7) Fix missing S/MIME header details that are needed to determine the type of S/MIME application
- v6.0.0, v1.1.19: Log rtf compression error, but otherwise ignore it and keep going and extract what we can.
**A big shout out to @dnault ([runtime javadoc](https://github.com/dnault/therapi-runtime-javadoc)), @remkop ([picocli](https://picocli.info/)) and @markenwerk
([S/MIME](https://github.com/markenwerk/java-utils-mail-smime) and [DKIM](https://github.com/markenwerk/java-utils-mail-dkim)) for working with me to make the
libraries work with JDK7+ and do what Simple Java Mail needed! Finally a great many thanks the numerous contributors on Simple Java Mail as well as
[outlook-message-parser](https://github.com/bbottema/outlook-message-parser) - this release would not be there without you.**
v5.5.0 - [v5.5.1](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C5.5.1%7Cjar)
- v5.5.1 (20-October-2019): [#230](https://github.com/bbottema/simple-java-mail/issues/230) Bugfix: Missing address value in address headers (ie. Return-Path) not handled properly, resulting in Exception
- v5.5.0 (15-October-2019): [#229](https://github.com/bbottema/simple-java-mail/issues/229) Bugfix: Timeouts not working for synchronous sendMail calls.
If you had connection properties configured for non-async send jobs, only now they will actually start to take effect.
[v5.4.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C5.4.0%7Cjar) (28-August-2019)
- [#221](https://github.com/bbottema/simple-java-mail/issues/221) API bugfix: server identity verification should not be tied to host trusting
- [#226](https://github.com/bbottema/simple-java-mail/issues/226) Bug fix: Attachments with spaces in name are not handled properly
- [#218](https://github.com/bbottema/simple-java-mail/issues/218) Enhancement: make Email serializable
- [#227](https://github.com/bbottema/simple-java-mail/issues/227) Enhancement: Make parsing recipients from EML file more lenient
- [#225](https://github.com/bbottema/simple-java-mail/issues/225) Enhancement: Clarify dependency on Jakarta Activation: DataSources no longer work on Java 9+
[v5.3.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C5.3.0%7Cjar) (16-August-2019)
- [#215](https://github.com/bbottema/simple-java-mail/issues/215) Bug: Current DKIM header canonicalization can lead to invalid DKIM
Note this release should have no impact, but nonetheless is a minor update so you can determine for yourself if this update would cause issues.
The release changes DKIM header canonicalization from SIMPLE to RELAXED.
[v5.2.1](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C5.2.1%7Cjar) (16-August-2019)
- [#219](https://github.com/bbottema/simple-java-mail/issues/219) Bug: MimeMessageParser rejects attachments with duplicate names
[v5.2.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C5.2.0%7Cjar) (7-July-2019)
- [#213](https://github.com/bbottema/simple-java-mail/issues/213) Update from javax.mail:1.6.0 to jakarta.mail:1.6.3
Note that dependencies that switched as well have been updated as part of this change. This includes the optional DKIM library and the email validation library:
- net.markenwerk:utils-mail-dkim (1.1.10 -> 1.2.0)
- com.github.bbottema:emailaddress-rfc2822 (1.1.2 -> 2.1.3)
v5.1.1 - [v5.1.7](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C5.1.7%7Cjar)
- v5.1.7 (22-May-2019): [#171](https://github.com/bbottema/simple-java-mail/issues/171) Header validation tripping on known safe emails due to References header
- v5.1.6 (27-April-2019): [#204](https://github.com/bbottema/simple-java-mail/issues/204) A Concurrent exception when an async process starts when the previous connection pool didn't shutdown in time
- v5.1.6 (27-April-2019): [#204](https://github.com/bbottema/simple-java-mail/issues/204) B Exceptions in threads are now caught and logged and don't bubble up anymore. Note that more comprehensive exception handling will be available in 6.0.0 ([#148](https://github.com/bbottema/simple-java-mail/issues/148)).
- v5.1.5 (24-April-2019): [#202](https://github.com/bbottema/simple-java-mail/issues/202) Fixed ConcurrentModificationException when moving invalid embedded images as regular attachments
- v5.1.4 (5-April-2019): [#163](https://github.com/bbottema/simple-java-mail/issues/163) Fixed missing mimetype for attachments when parsing Outlook messages where mimeTag was not included
- v5.1.3 (15-Januari-2019): Updated to newer rfc-validator version, which fixed a regression bug in that library
- v5.1.2 (9-Januari-2019): [#189](https://github.com/bbottema/simple-java-mail/issues/189) Bugfix for missing timeout config for .testConnection() function
- v5.1.1 (22-December-2018): [#190](https://github.com/bbottema/simple-java-mail/issues/190) Fix for transitive dependency clash because of emailaddress-rfc2822 library
[v5.1.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C5.1.0%7Cjar) (21-November-2018)
- [#179](https://github.com/bbottema/simple-java-mail/issues/179) You can now [test the connection](https://www.simplejavamail.org/features.html#section-connection-test) to the SMTP server
v5.0.1 - [v5.0.8](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C5.0.8%7Cjar)
- v5.0.8 (27-Oktober-2018): [#178](https://github.com/bbottema/simple-java-mail/issues/178) Fix the annoying vulnerability Github report about spring-core
- v5.0.7 (27-Oktober-2018): [#175](https://github.com/bbottema/simple-java-mail/issues/175) Attachment names are not always parsed properly from MimeMessage
- v5.0.6 (3-Oktober-2018): [#167](https://github.com/bbottema/simple-java-mail/issues/167) Email addresses validated despite cleared validation validation criteria
- v5.0.5 (3-Oktober-2018): [#137](https://github.com/bbottema/simple-java-mail/issues/137) When replying to an email with HTML, the result body is empty
- v5.0.4 (22-September-2018): [#168](https://github.com/bbottema/simple-java-mail/issues/168) Properties aquired through ConfigLoader should be typed explicitly and converted if necessary
- v5.0.3 (11-April-2018): [#136](https://github.com/bbottema/simple-java-mail/issues/136) ServerConfig class should be public API
- v5.0.2 (7-April-2018): [#135](https://github.com/bbottema/simple-java-mail/issues/135) trustingAllHosts should be public on the Builder API
- v5.0.2 (7-April-2018): [#131](https://github.com/bbottema/simple-java-mail/issues/131) NamedDataSource should implement EncodingAware
- v5.0.1 (10-March-2018): [#130](https://github.com/bbottema/simple-java-mail/issues/130) java.lang.ClassNotFoundException: net.markenwerk.utils.mail.dkim.DkimMessage. Solves the issue of missing optional class DKIM even when not used
[v5.0.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C5.0.0%7Cjar) (14-Februari-2018)
Also see the [migration notes](https://www.simplejavamail.org/migration-notes-5.0.0.html#navigation)
#### New features ####
- [#116](https://github.com/bbottema/simple-java-mail/issues/116) You can now test the connection to the SMTP server
- [#115](https://github.com/bbottema/simple-java-mail/issues/115) Create mailers with a very robust MailerBuilder API, able to ignore defaults as well
- [#114](https://github.com/bbottema/simple-java-mail/issues/114) Create emails with a very robust EmailBuilder API, able to ignore defaults as well. Now includes support for InternetAddress. Also copy emails.
- [#107](https://github.com/bbottema/simple-java-mail/issues/107) You can now easily forward or reply to emails!
#### Security updates ####
- [#111](https://github.com/bbottema/simple-java-mail/issues/111) Protocol properties for SMTPS are now applied properly
- [#105](https://github.com/bbottema/simple-java-mail/issues/105) SMTP tries to upgrade to TLS while SMTP_TLS now enforces it and for both SMTP_TLS and SMTPS, [mail.smtp.ssl.checkserveridentity](https://javaee.github.io/javamail/docs/api/com/sun/mail/smtp/package-summary.html) is set to true
#### Maintenance updates ####
Complete [Javadoc](https://www.javadoc.io/doc/org.simplejavamail/simple-java-mail) overhaul. Navigating the Javadoc should be much more consistent now (builder API being the single *public* source of truth).
- [#122](https://github.com/bbottema/simple-java-mail/issues/122) The email-rfc2822-validator library has been made a proper Maven dependency (not packaged along anymore)
- [#120](https://github.com/bbottema/simple-java-mail/issues/120) The DKIM library has been made an optional proper Maven dependency (not packaged along anymore)
- [#119](https://github.com/bbottema/simple-java-mail/issues/119) Switched optional Spring dependency version to property and now testing with 4.3.11.RELEASE
- [#113](https://github.com/bbottema/simple-java-mail/issues/113) Updated the underlying JavaMail to 1.6.0
#### Bugfixes ####
- [#110](https://github.com/bbottema/simple-java-mail/issues/110) Trusted hosts should be space-delimited
- [#109](https://github.com/bbottema/simple-java-mail/issues/109) Email headers should be allowed to be empty (now conversion errors can occur as well)
- [#103](https://github.com/bbottema/simple-java-mail/issues/103) Converting to MimeMessage results in an invalid Content-Disposition for attachments
[v4.4.5](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C4.4.5%7Cjar) (2-September-2017)
- [#101](https://github.com/bbottema/simple-java-mail/issues/101) API backwards compatibility update, reinstate old addRecipient API as deprecated (sorry for removing it abruptly)
[v4.4.4](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C4.4.4%7Cjar) (23-August-2017)
API usability release. **This relase streamlined the recipient setters, breaking backwards compatibility (but straightforward to fix)**
- [#95](https://github.com/bbottema/simple-java-mail/issues/95) Feature: Add support native API for [setting Return-Receipt-To](https://www.simplejavamail.org/features.html#section-return-receipt) header
- [#93](https://github.com/bbottema/simple-java-mail/issues/93) Feature: Add support native API for setting [Disposition-Notification-To](https://www.simplejavamail.org/features.html#section-return-receipt) header
- [#91](https://github.com/bbottema/simple-java-mail/issues/91) **Feature: Add support for parsing [preformatted email addresses](https://www.simplejavamail.org/features.html#section-add-recipients) that include both name and address**
- [#94](https://github.com/bbottema/simple-java-mail/issues/94) Bugfix: A single EmailBuilder would build emails that all share the same collections for recipients, attachments and embedded images
- [#98](https://github.com/bbottema/simple-java-mail/issues/98) Bugfix: Subject and body content should be optional
[v4.3.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C4.3.0%7Cjar) (12-August-2017)
Security and timeout release.
This version safeguards against SMTP injection attack from external values entering the library through *Email* instance. Also, this release introduces default/configurable timeouts for connecting, reading and writing when sending an email.
- [#89](https://github.com/bbottema/simple-java-mail/issues/89) Support multiple delimited recipient addresses sharing the same TO/CC/BCC name
- [#88](https://github.com/bbottema/simple-java-mail/issues/88) **Safeguard subject property (and others) against SMTP CRLF injection attacks**
- [#85](https://github.com/bbottema/simple-java-mail/issues/85) **Apply configurable timeouts when sending emails**
- [#83](https://github.com/bbottema/simple-java-mail/issues/83) Parse INLINE attachments without ID as regular attachments when converting (mostly applicable to Apple emails)
[v4.2.3](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C4.2.3%7Cjar) (21-May-2017)
- [#79](https://github.com/bbottema/simple-java-mail/issues/79): Enhancement: define custom message ID on the Email object
- [#74](https://github.com/bbottema/simple-java-mail/issues/74): v4.2.3-java-6-release: A java6 version with limited capabilities:
I've released a customised java6 release with a customised outlook-message-parser 1.1.16-java6-release. **This is the last java6 release** I will do, as it is simply too much manual labor to create a limited second edition.
For this edition, I've removed the JDK7 Phaser completely which has the following consequences:
- If authenticated proxy is used, the bridging proxy server will not be shut down automatically (and might not run the second time)
- If mails are sent in async mode, the connection pool will not be shut down anymore by itself
This means your server/application might not stop properly due to lingering processes. To be completely safe, only send emails in sync mode (used by default) and don't use authenticated proxy config.
[v4.2.2](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C4.2.2%7Cjar) (10-May-2017)
- [#73](https://github.com/bbottema/simple-java-mail/issues/73): Patch: fix for sending emails in async mode, which makes sure the connection pool is always closed when the last *known* email has been sent. Without this fix, the connection pool keeps any parent process running (main thread or Tomcat for example) until a hard kill.
[v4.2.1](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C4.2.1%7Cjar) (12-Feb-2017)
Patch: streamlined convenience methods for adding recipients.
[v4.2.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C4.2.0%7Cjar) (12-Feb-2017)
**Major feature: Using the EmailConverter you can now [convert between](https://www.simplejavamail.org/features.html#section-converting) Outlook .msg, EML, MimeMessage and Email**!
- [#66](https://github.com/bbottema/simple-java-mail/issues/66): Feature: [convert](https://www.simplejavamail.org/features.html#section-converting) email to EML
- [#65](https://github.com/bbottema/simple-java-mail/issues/65): Feature: [read outlook messages](https://www.simplejavamail.org/modules.html#outlook-module) from .msg file
- [#64](https://github.com/bbottema/simple-java-mail/issues/64): **Feature: Added support for [logging-only mode](https://www.simplejavamail.org/debugging.html#section-debug-mode) that skips the actual sending of emails**
- [#63](https://github.com/bbottema/simple-java-mail/issues/63): Feature: Already including in previous patch update: [Spring support](https://www.simplejavamail.org/modules.html#spring-module) (read properties from Spring context)
- [#69](https://github.com/bbottema/simple-java-mail/issues/69): Enhancement: Expanded EmailBuilder API to inlude more options for setting (multiple) recipients
- [#70](https://github.com/bbottema/simple-java-mail/issues/70): Enhancement: Most public API now have defensive null-checks for required fields (Fail Fast support)
- [#68](https://github.com/bbottema/simple-java-mail/issues/68): Bugfix: Name should be required for embedded images (added safeguards)
- [#67](https://github.com/bbottema/simple-java-mail/issues/67): Bugfix: Error when name was omitted for attachment
- minor: added methods on AttachmentResource that reads back the content as (encoded) String
- other: internal testing is now done using Wiser SMTP test server for testing live sending emails
**Note**: Starting this release, there will always be a Java6 compatible release as well versioned: "x.y.z-java6-release"
[v4.1.3](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C4.1.3%7Cjar) (28-Jan-2017)
- [#61](https://github.com/bbottema/simple-java-mail/issues/61): Feature: Add support for [providing your own Properties](https://www.simplejavamail.org/features.html#section-custom-properties) object
- [#63](https://github.com/bbottema/simple-java-mail/issues/63): **Feature: [Spring support](https://www.simplejavamail.org/modules.html#spring-module) (read properties from Spring context)**
- [#58](https://github.com/bbottema/simple-java-mail/issues/58): Bugfix: Add support for non-English attachment and embedded image names
- [#62](https://github.com/bbottema/simple-java-mail/issues/62): Bugfix: Empty properties loaded from config should be considered null
**NOTE**: ConfigLoader moved from `/internal/util` to `/util`
[v4.1.2](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C4.1.2%7Cjar) (07-Nov-2016)
- [#52](https://github.com/bbottema/simple-java-mail/issues/52): bug fix for windows / linux disparity when checking socket status
- [#56](https://github.com/bbottema/simple-java-mail/issues/56): bug fix for IOException when signing dkim with a File reference
[v4.1.1](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C4.1.1%7Cjar) (30-Jul-2016)
- [#50](https://github.com/bbottema/simple-java-mail/issues/50): bug fix for manual naming datasources
[v4.1.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C4.1.0%7Cjar) (22-Jul-2016)
- [#48](https://github.com/bbottema/simple-java-mail/issues/48): Added programmatic support trusting hosts for SSL connections
- [#47](https://github.com/bbottema/simple-java-mail/issues/47): Honor given names, deduce extension from datasource name, and more robust support for parsing mimemessages
[v4.0.0](https://search.maven.org/#artifactdetails%7Corg.simplejavamail%7Csimple-java-mail%7C4.0.0%7Cjar) (05-Jul-2016)
- [#41](https://github.com/bbottema/simple-java-mail/issues/41): added support for fast parallel batch processing
- [#42](https://github.com/bbottema/simple-java-mail/issues/42): **added support for config files**
- [#43](https://github.com/bbottema/simple-java-mail/issues/43): removed logging implementation dependencies from distribution and documented various sample configs
- [#39](https://github.com/bbottema/simple-java-mail/issues/39): simplified and renamed packages to reflect the domain name of the new website: [simplejavamail.org](https://www.simplejavamail.org)
- [#38](https://github.com/bbottema/simple-java-mail/issues/38): added support for anonymous proxy
- [#38](https://github.com/bbottema/simple-java-mail/issues/38): **added support for authenticated proxy**
**NOTE**: All packages have been renamed to "org.simplejavamail.(..)"
**NOTE**: Switched to Java 7
[v3.1.1](https://search.maven.org/#artifactdetails%7Corg.codemonkey.simplejavamail%7Csimple-java-mail%7C3.1.1%7Cjar) (11-May-2016)
**Major feature: DKIM support**!
- [#36](https://github.com/bbottema/simple-java-mail/issues/36): Added proper toString and equals methods for the Email classes
- [#33](https://github.com/bbottema/simple-java-mail/issues/33): Added [support for DKIM](https://www.simplejavamail.org/features.html#section-dkim) domain key signing
*NOTE*: this is the last release still using Java 6. Next release will be using Java 7.
/edit: starting with 4.2.0 every release will now have a "x.y.z-java6-release" release as well
[v3.0.2](https://search.maven.org/#artifactdetails%7Corg.codemonkey.simplejavamail%7Csimple-java-mail%7C3.0.2%7Cjar) (07-May-2016)
- [#35](https://github.com/bbottema/simple-java-mail/issues/35): added proper .equals() and .toString() methods
- [#34](https://github.com/bbottema/simple-java-mail/issues/34): Fixed bug when disposition is missing (assume it is an attachment)
- other: added findbugs support internally
[v3.0.1](https://search.maven.org/#artifactdetails%7Corg.codemonkey.simplejavamail%7Csimple-java-mail%7C3.0.1%7Cjar) (29-Feb-2016)
* [#31](https://github.com/bbottema/simple-java-mail/issues/31): Fixed EmailAddressCriteria.DEFAULT and clarified Javadoc
[v3.0.0](https://search.maven.org/#artifactdetails%7Corg.codemonkey.simplejavamail%7Csimple-java-mail%7C3.0.0%7Cjar) (26-Feb-2016)
* [#30](https://github.com/bbottema/simple-java-mail/issues/30): Improved the demonstration class to include attachments and embedded images
* [#29](https://github.com/bbottema/simple-java-mail/issues/29): The package has been restructured for future maintenance, breaking backwards compatibility
* [#28](https://github.com/bbottema/simple-java-mail/issues/28): Re-added improved email validation facility
* [#22](https://github.com/bbottema/simple-java-mail/issues/22): Added conversion to and from MimeMessage. You can now consume and produce MimeMessage objects with simple-java-mail
[v2.5.1](https://search.maven.org/#artifactdetails%7Corg.codemonkey.simplejavamail%7Csimple-java-mail%7C2.5.1%7Cjar) (19-Jan-2016)
* [#25](https://github.com/bbottema/simple-java-mail/issues/25): Added finally clause that will always close socket properly in case of an exception
[v2.5](https://search.maven.org/#artifactdetails%7Corg.codemonkey.simplejavamail%7Csimple-java-mail%7C2.5%7Cjar) (19-Jan-2016)
* [#24](https://github.com/bbottema/simple-java-mail/issues/24): Updated dependencies SLF4J to 1.7.13 and switched to the updated javax mail package com.sun.mail:javax.mail 1.5.5
[v2.4](https://search.maven.org/#artifactdetails%7Corg.codemonkey.simplejavamail%7Csimple-java-mail%7C2.4%7Cjar) (12-Aug-2015)
* [#21](https://github.com/bbottema/simple-java-mail/issues/21): builder API uses CC and BCC recipient types incorrectly
[v2.3](https://search.maven.org/#artifactdetails%7Corg.codemonkey.simplejavamail%7Csimple-java-mail%7C2.3%7Cjar) (21-Jul-2015)
* [#19](https://github.com/bbottema/simple-java-mail/issues/19): supporting custom Session Properties now and emergency access to internal Session object.
[v2.2](https://search.maven.org/#artifactdetails%7Corg.codemonkey.simplejavamail%7Csimple-java-mail%7C2.2%7Cjar) (09-May-2015)
* [#3](https://github.com/bbottema/simple-java-mail/issues/3): turned off email regex validation by default, with the option to turn it back on
* [#7](https://github.com/bbottema/simple-java-mail/issues/7): fixed NullPointerException when using your own Session instance
* [#10](https://github.com/bbottema/simple-java-mail/issues/10): properly UTF-8 encode recipient addresses
* [#14](https://github.com/bbottema/simple-java-mail/issues/14): switched to [SLF4J](https://www.slf4j.org/), so you can easily use your own selected logging framework
* [#17](https://github.com/bbottema/simple-java-mail/issues/17): Added [fluent interface](https://en.wikipedia.org/wiki/Builder_pattern) for building emails (see [here](https://www.simplejavamail.org/#section-builder-api) for an example)
[v2.1](https://search.maven.org/#artifactdetails%7Corg.codemonkey.simplejavamail%7Csimple-java-mail%7C2.1%7Cjar) (09-Aug-2012)
* fixed character encoding for reply-to, from, to, body text and headers (to UTF-8)
* fixed bug where Recipient was not public resulting in uncompilable code when calling email.getRecipients()
[v2.0](https://search.maven.org/#artifactdetails%7Corg.codemonkey.simplejavamail%7Csimple-java-mail%7C2.0%7Cjar) (20-Aug-2011)
* added support for adding open headers, such as 'X-Priority: 2'
[v1.9.1](https://search.maven.org/#artifactdetails%7Corg.codemonkey.simplejavamail%7Csimple-java-mail%7C1.9.1%7Cjar) (08-Aug-2011)
* updated for Maven support
v1.9 (6-Aug-2011)
* added support for JavaMail's reply-to address
* made port optional as to support port defaulting based on protocol
* added transport strategy default in the createSession method
* tightened up thrown exceptions (MailException instead of RuntimeException)
* added and fixed [Javadoc](https://www.javadoc.io/doc/org.simplejavamail/simple-java-mail)
v1.8
* Added support for TLS (tested with gmail)
v1.7 (22-Mar-2011)
Added support for SSL! (tested with gmail)
* improved argument validation when creating a Mailer without preconfigured Session instance
known possible issue: SSL self-signed certificates might not work (yet). Please let me know by e-mail or create a new issue
v1.6
Completed migration to Java Simple Mail project.
* removed all Vesijama references
* updated TestMail demonstration class for clarification
* updated readme.txt for test run instructions
* included log4j.properties
v1.4 (15-Jan-2011)
vX.X (26-Apr-2009)
* Initial upload to Google Code.
| 0 |
dain/leveldb | Port of LevelDB to Java | null | # LevelDB in Java
This is a rewrite (port) of [LevelDB](http://code.google.com/p/leveldb/) in
Java. This goal is to have a feature complete implementation that is within
10% of the performance of the C++ original and produces byte-for-byte exact
copies of the C++ code.
# Current status
Currently the code base is basically functional, but only trivially tested.
In some places, this code is a literal conversion of the C++ code and in
others it has been converted to a more natural Java style. The plan is to
leave the code closer to the C++ original until the baseline performance has
been established.
## API Usage:
Recommended Package imports:
```java
import org.iq80.leveldb.*;
import static org.iq80.leveldb.impl.Iq80DBFactory.*;
import java.io.*;
```
Opening and closing the database.
```java
Options options = new Options();
options.createIfMissing(true);
DB db = factory.open(new File("example"), options);
try {
// Use the db in here....
} finally {
// Make sure you close the db to shutdown the
// database and avoid resource leaks.
db.close();
}
```
Putting, Getting, and Deleting key/values.
```java
db.put(bytes("Tampa"), bytes("rocks"));
String value = asString(db.get(bytes("Tampa")));
db.delete(bytes("Tampa"), wo);
```
Performing Batch/Bulk/Atomic Updates.
```java
WriteBatch batch = db.createWriteBatch();
try {
batch.delete(bytes("Denver"));
batch.put(bytes("Tampa"), bytes("green"));
batch.put(bytes("London"), bytes("red"));
db.write(batch);
} finally {
// Make sure you close the batch to avoid resource leaks.
batch.close();
}
```
Iterating key/values.
```java
DBIterator iterator = db.iterator();
try {
for(iterator.seekToFirst(); iterator.hasNext(); iterator.next()) {
String key = asString(iterator.peekNext().getKey());
String value = asString(iterator.peekNext().getValue());
System.out.println(key+" = "+value);
}
} finally {
// Make sure you close the iterator to avoid resource leaks.
iterator.close();
}
```
Working against a Snapshot view of the Database.
```java
ReadOptions ro = new ReadOptions();
ro.snapshot(db.getSnapshot());
try {
// All read operations will now use the same
// consistent view of the data.
... = db.iterator(ro);
... = db.get(bytes("Tampa"), ro);
} finally {
// Make sure you close the snapshot to avoid resource leaks.
ro.snapshot().close();
}
```
Using a custom Comparator.
```java
DBComparator comparator = new DBComparator(){
public int compare(byte[] key1, byte[] key2) {
return new String(key1).compareTo(new String(key2));
}
public String name() {
return "simple";
}
public byte[] findShortestSeparator(byte[] start, byte[] limit) {
return start;
}
public byte[] findShortSuccessor(byte[] key) {
return key;
}
};
Options options = new Options();
options.comparator(comparator);
DB db = factory.open(new File("example"), options);
```
Disabling Compression
```java
Options options = new Options();
options.compressionType(CompressionType.NONE);
DB db = factory.open(new File("example"), options);
```
Configuring the Cache
```java
Options options = new Options();
options.cacheSize(100 * 1048576); // 100MB cache
DB db = factory.open(new File("example"), options);
```
Getting approximate sizes.
```java
long[] sizes = db.getApproximateSizes(new Range(bytes("a"), bytes("k")), new Range(bytes("k"), bytes("z")));
System.out.println("Size: "+sizes[0]+", "+sizes[1]);
```
Getting database status.
```java
String stats = db.getProperty("leveldb.stats");
System.out.println(stats);
```
Getting informational log messages.
```java
Logger logger = new Logger() {
public void log(String message) {
System.out.println(message);
}
};
Options options = new Options();
options.logger(logger);
DB db = factory.open(new File("example"), options);
```
Destroying a database.
```java
Options options = new Options();
factory.destroy(new File("example"), options);
```
# Projects using this port of LevelDB
* [ActiveMQ Apollo](http://activemq.apache.org/apollo/): Defaults to using leveldbjni, but falls
back to this port if the jni port is not available on your platform.
| 0 |
bastillion-io/Bastillion | Bastillion is a web-based SSH console that centrally manages administrative access to systems. Web-based administration is combined with management and distribution of user's public SSH keys. | bastion-host java javascript ssh ssh-client ssh-key ssh-server web-app web-based | 

 Bastillion
======
Bastillion is a web-based SSH console that centrally manages administrative access to systems. Web-based administration is combined with management and distribution of user's public SSH keys. Key management and administration is based on profiles assigned to defined users.
Administrators can login using two-factor authentication with [Authy](https://authy.com/) or [Google Authenticator](https://github.com/google/google-authenticator). From there they can manage their public SSH keys or connect to their systems through a web-shell. Commands can be shared across shells to make patching easier and eliminate redundant command execution.
Bastillion layers TLS/SSL on top of SSH and acts as a bastion host for administration. Protocols are stacked (TLS/SSL + SSH) so infrastructure cannot be exposed through tunneling / port forwarding. More details can be found in the following whitepaper: [Implementing a Trusted Third-Party System for Secure Shell](https://www.bastillion.io/docs/using/whitepaper). Also, SSH key management is enabled by default to prevent unmanaged public keys and enforce best practices.
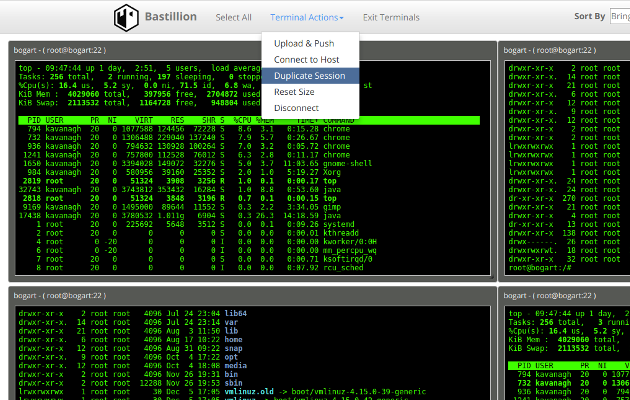
Bastillion Releases
------
Bastillion is available for free use under the Prosperity Public License
https://github.com/bastillion-io/Bastillion/releases
or purchase from the AWS marketplace
https://aws.amazon.com/marketplace/pp/Loophole-LLC-Bastillion/B076PNFPCL
Also, Bastillion can be installed on FreeBSD via the FreeBSD ports system. To install via the binary package, simply run:
pkg install security/bastillion
Prerequisites
-------------
**Open-JDK / Oracle-JDK - 1.9 or greater**
*apt-get install openjdk-9-jdk*
> http://www.oracle.com/technetwork/java/javase/downloads/index.html
**Install [Authy](https://authy.com/) or [Google Authenticator](https://github.com/google/google-authenticator)** to enable two-factor authentication with Android or iOS
| Application | Android | iOS |
|----------------------|-----------------------------------------------------------------------------------------------------|----------------------------------------------------------------------------|
| Authy | [Google Play](https://play.google.com/store/apps/details?id=com.authy.authy) | [iTunes](https://itunes.apple.com/us/app/authy/id494168017) |
| Google Authenticator | [Google Play](https://play.google.com/store/apps/details?id=com.google.android.apps.authenticator2) | [iTunes](https://itunes.apple.com/us/app/google-authenticator/id388497605) |
To Run Bundled with Jetty
------
Download bastillion-jetty-vXX.XX.tar.gz
https://github.com/bastillion-io/Bastillion/releases
Export environment variables
for Linux/Unix/OSX
export JAVA_HOME=/path/to/jdk
export PATH=$JAVA_HOME/bin:$PATH
for Windows
set JAVA_HOME=C:\path\to\jdk
set PATH=%JAVA_HOME%\bin;%PATH%
Start Bastillion
for Linux/Unix/OSX
./startBastillion.sh
for Windows
startBastillion.bat
More Documentation at: https://www.bastillion.io/docs/index.html
Build from Source
------
Install Maven 3 or greater
*apt-get install maven*
> http://maven.apache.org
Export environment variables
export JAVA_HOME=/path/to/jdk
export M2_HOME=/path/to/maven
export PATH=$JAVA_HOME/bin:$M2_HOME/bin:$PATH
In the directory that contains the pom.xml run
mvn package jetty:run
*Note: Doing a mvn clean will delete the H2 DB and wipe out all the data.*
Using Bastillion
------
Open browser to https://\<whatever ip\>:8443
Login with
username:admin
password:changeme
*Note: When using the AMI instance, the password is defaulted to the \<Instance ID\>. Also, the AMI uses port 443 as in https://\<Instance IP\>:443*
Managing SSH Keys
------
By default Bastillion will overwrite all values in the specified authorized_keys file for a system. You can disable key management by editing BastillionConfig.properties file and use Bastillion only as a bastion host. This file is located in the jetty/bastillion/WEB-INF/classes directory. (or the src/main/resources directory if building from source)
#set to false to disable key management. If false, the Bastillion public key will be appended to the authorized_keys file (instead of it being overwritten completely).
keyManagementEnabled=false
Also, the authorized_keys file is updated/refreshed periodically based on the relationships defined in the application. If key management is enabled the refresh interval can be specified in the BastillionConfig.properties file.
#authorized_keys refresh interval in minutes (no refresh for <=0)
authKeysRefreshInterval=120
By default Bastillion will generated and distribute the SSH keys managed by administrators while having them download the generated private. This forces admins to use strong passphrases for keys that are set on systems. The private key is only available for download once and is not stored on the application side. To disable and allow administrators to set any public key edit the BastillionConfig.properties.
#set to true to generate keys when added/managed by users and enforce strong passphrases set to false to allow users to set their own public key
forceUserKeyGeneration=false
Supplying a Custom SSH Key Pair
------
Bastillion generates its own public/private SSH key upon initial startup for use when registering systems. You can specify a custom SSH key pair in the BastillionConfig.properties file.
For example:
#set to true to regenerate and import SSH keys --set to true
resetApplicationSSHKey=true
#SSH Key Type 'dsa' or 'rsa'
sshKeyType=rsa
#private key --set pvt key
privateKey=/Users/kavanagh/.ssh/id_rsa
#public key --set pub key
publicKey=/Users/kavanagh/.ssh/id_rsa.pub
#default passphrase --leave blank if passphrase is empty
defaultSSHPassphrase=myPa$$w0rd
After startup and once the key has been registered it can then be removed from the system. The passphrase and the key paths will be removed from the configuration file.
Adjusting Database Settings
------
Database settings can be adjusted in the configuration properties.
#Database user
dbUser=bastillion
#Database password
dbPassword=p@$$w0rd!!
#Database JDBC driver
dbDriver=org.h2.Driver
#Connection URL to the DB
dbConnectionURL=jdbc:h2:keydb/bastillion;CIPHER=AES;
By default the datastore is set as embedded, but a remote H2 database can supported through adjusting the connection URL.
#Connection URL to the DB
dbConnectionURL=jdbc:h2:tcp://<host>:<port>/~/bastillion;CIPHER=AES;
External Authentication
------
External Authentication can be enabled through the BastillionConfig.properties.
For example:
#specify a external authentication module (ex: ldap-ol, ldap-ad). Edit the jaas.conf to set connection details
jaasModule=ldap-ol
Connection details need to be set in the jaas.conf file
ldap-ol {
com.sun.security.auth.module.LdapLoginModule SUFFICIENT
userProvider="ldap://hostname:389/ou=example,dc=bastillion,dc=com"
userFilter="(&(uid={USERNAME})(objectClass=inetOrgPerson))"
authzIdentity="{cn}"
useSSL=false
debug=false;
};
Administrators will be added as they are authenticated and profiles of systems may be assigned by full-privileged users.
User LDAP roles can be mapped to profiles defined in Bastillion through the use of the org.eclipse.jetty.jaas.spi.LdapLoginModule.
ldap-ol-with-roles {
//openldap auth with roles that can map to profiles
org.eclipse.jetty.jaas.spi.LdapLoginModule required
debug="false"
useLdaps="false"
contextFactory="com.sun.jndi.ldap.LdapCtxFactory"
hostname="<SERVER>"
port="389"
bindDn="<BIND-DN>"
bindPassword="<BIND-DN PASSWORD>"
authenticationMethod="simple"
forceBindingLogin="true"
userBaseDn="ou=users,dc=bastillion,dc=com"
userRdnAttribute="uid"
userIdAttribute="uid"
userPasswordAttribute="userPassword"
userObjectClass="inetOrgPerson"
roleBaseDn="ou=groups,dc=bastillion,dc=com"
roleNameAttribute="cn"
roleMemberAttribute="member"
roleObjectClass="groupOfNames";
};
Users will be added/removed from defined profiles as they login and when the role name matches the profile name.
Auditing
------
Auditing is disabled by default. Audit logs can be enabled through the **log4j2.xml** by uncommenting the **io.bastillion.manage.util.SystemAudit** and the **audit-appender** definitions.
> https://github.com/bastillion-io/Bastillion/blob/master/src/main/resources/log4j2.xml#L19-L22
Auditing through the application is only a proof of concept. It can be enabled in the BastillionConfig.properties.
#enable audit --set to true to enable
enableInternalAudit=true
Screenshots
-----------
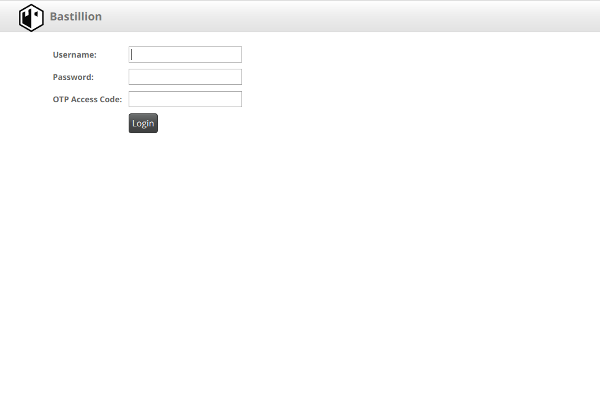
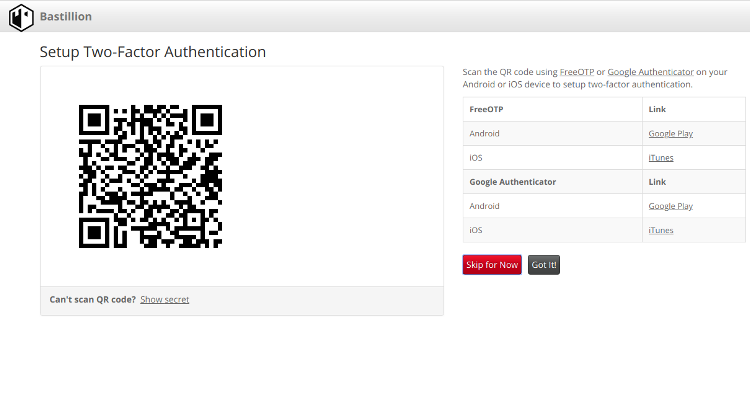
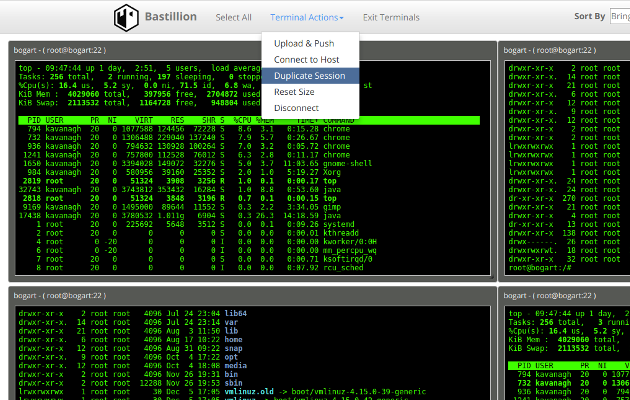
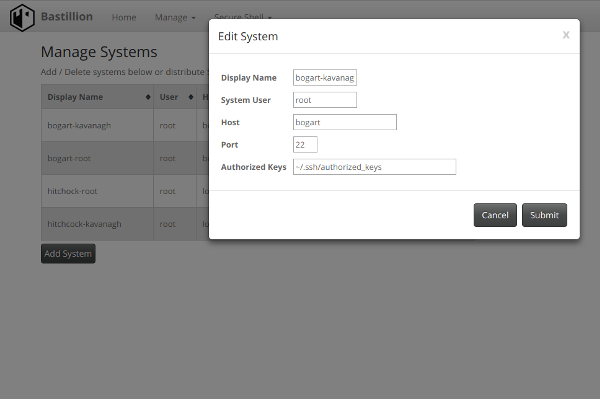
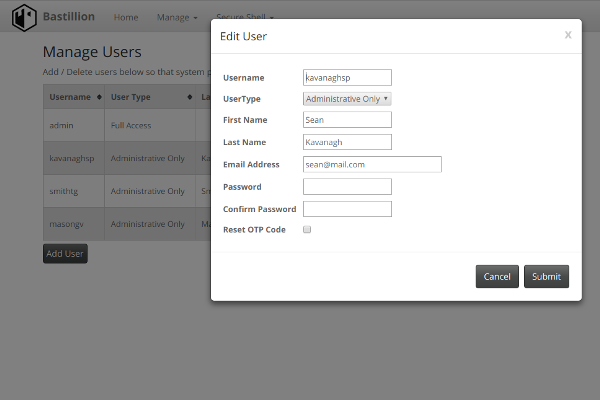
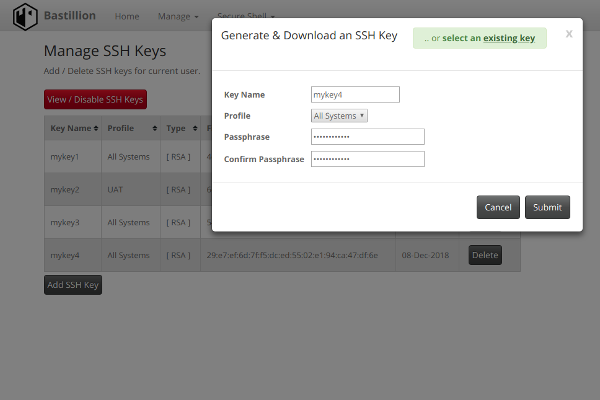
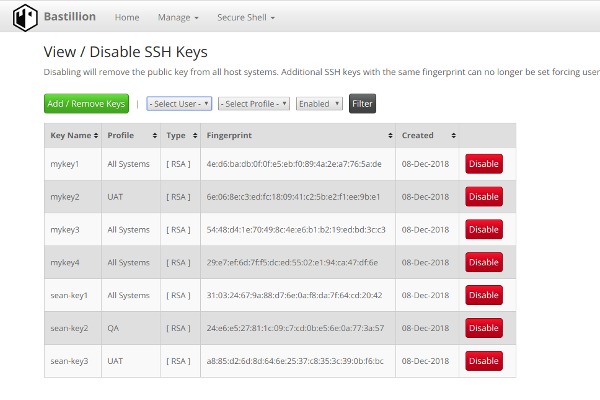
Acknowledgments
------
Special thanks goes to these amazing projects which makes this (and other great projects) possible.
+ [JSch](http://www.jcraft.com/jsch) Java Secure Channel - by [ymnk](https://github.com/ymnk)
+ [term.js](https://github.com/chjj/term.js) A terminal written in javascript - by [chjj](https://github.com/chjj)
Third-party dependencies are mentioned in the [_3rdPartyLicenses.md_](3rdPartyLicenses.md)
The Prosperity Public License
-----------
Bastillion is available for use under the Prosperity Public License
Author
------
**Loophole, LLC - Sean Kavanagh**
+ sean.p.kavanagh6@gmail.com
+ https://twitter.com/spkavanagh6
| 0 |
cbeust/jcommander | Command line parsing framework for Java | null | null | 0 |
geometer/FBReaderJ | Official FBReaderJ project repository | null | null | 0 |
binarywang/weixin-java-miniapp-demo | 基于Spring Boot 和 WxJava 实现的微信小程序Java后端Demo | weapp-demo wechat wechat-app wechat-mini-program wechat-sdk wechat-weapp weixin wx wxapp | [](https://gitee.com/binary/weixin-java-miniapp-demo)
[](https://github.com/binarywang/weixin-java-miniapp-demo)
[](https://travis-ci.org/binarywang/weixin-java-miniapp-demo)
-----------------------
<table align="center" cellspacing="0" cellpadding="0">
<tbody>
<tr>
<td align="left" valign="middle">
<a href="http://mp.weixin.qq.com/mp/homepage?__biz=MzI3MzAwMzk4OA==&hid=1&sn=f31af3bf562b116b061c9ab4edf70b61&scene=18#wechat_redirect" target="_blank">
<img height="120" src="https://gitee.com/binary/weixin-java-tools/raw/develop/images/qrcodes/mp.png">
</a>
</td>
<td align="center" valign="middle">
<a href="https://promotion.aliyun.com/ntms/act/qwbk.html?userCode=7makzf5h" target="_blank">
<img height="120" src="https://gitee.com/binary/weixin-java-tools/raw/develop/images/banners/aliyun.jpg">
</a>
</td>
</tr>
</tbody>
</table>
### 本项目为WxJava的Demo演示程序,基于Spring Boot构建,实现微信小程序后端开发功能,支持多个小程序。
更多信息请查阅:https://github.com/Wechat-Group/WxJava
## 使用步骤:
1. **请注意,本demo为简化代码编译时加入了lombok支持,如果不了解lombok的话,请先学习下相关知识,比如可以阅读[此文章](https://mp.weixin.qq.com/s/cUc-bUcprycADfNepnSwZQ)**;
1. 另外,新手遇到问题,请务必先阅读[【开发文档首页】](https://github.com/Wechat-Group/WxJava/wiki)的常见问题部分,可以少走很多弯路,节省不少时间。
1. 配置:复制 `/src/main/resources/application.yml.template` 或者修改其扩展名生成 `application.yml` 文件,根据自己需要填写相关配置(需要注意的是:yml文件内的属性冒号后面的文字之前需要加空格,可参考已有配置,否则属性会设置不成功);
1. 运行Java程序:`WxMaDemoApplication`;
1. 如果需要接入消息服务,请配置微信小程序中的消息服务器地址:http://外网可访问的域名/wx/portal/{appid} (注意{appid}要使用对应的小程序的appid进行替换), 官方文档接入指引:https://developers.weixin.qq.com/miniprogram/dev/framework/server-ability/message-push.html
| 0 |
chaychan/TouTiao | 精仿今日头条 | null | # 精仿今日头条
精仿今日头条,数据是抓取今日头条App的数据。使用RxJava + Retrofit + MVP开发的开源项目,仅供学习用途。觉得对你有帮助的话请帮忙star一下,让更多人知道,多谢啦!
### 2022-11-09
  近期有个小伙伴向我反馈项目下下来后编译出错,愿意付费希望我解决下,今天抽空把问题解决了下,希望可以帮到他,当然也是无偿的,这个
项目从17年到现在已经5年了,很高兴还可以帮到一些刚入门的安卓朋友或者参考开发的朋友,由衷感谢大家支持和Star。
### 2019-12-02
  时间过得真快,不知不觉就过了一年,去年年底我更新了视频解析的逻辑,然后一直用到前段时间,有小伙伴issue上提出又不能解析了。由于前段时间比较忙,一直没能腾出时间来解决这个问题,上周末通过分析,发现以往解析视频的逻辑已经用不了了,所以重新找了个可以解析头条视频的网站,并分析到了他们的解析接口规则,修复了视频播放的问题,小伙伴们可以用作交流,讨论下,但不可用作商用,主要有以下两点原因:
- 调用解析接口并未获得该网站的同意,属于非正常渠道获取,仅用于学习交流
- 该网站可能变动接口规则,如果真的有解析视频方面的需求,可以和该网站联系和合作
### 2018-12-04 更新内容:
  项目已经发布很久了,最近看到有不少小伙伴帮我star,想想自己已经好久没有维护这个项目了,主要是因为忙,最近抽空把issue上提出的问题解决了,方便需要参考到其中某些功能的小伙伴使用,同时也希望这个项目可以成为我在github上首个star破k的项目,希望小伙伴们可以帮忙star一下,多谢了,项目的话我也会继续维护和更新。
- 更改右滑关闭的依赖库,解决8.0以上系统不兼容右滑的问题;
- 修改视频解析逻辑,之前通过加载详情页成功后延迟5秒获取html中的video标签内的视频地址,体验极差,故花了几天时间寻找解析头条视频地址的方法,经过多次实验终于成功;
- 解决视频播放错乱的问题;更换了最新版的饺子播放器;
- 整理项目结构,包的路径等
# Apk下载地址
[[点击下载体验](https://raw.githubusercontent.com/chaychan/TouTiao/master/apk/news.apk)]
扫码下载体验:

# Blog
[http://blog.csdn.net/chay_chan/article/details/75319452](http://blog.csdn.net/chay_chan/article/details/75319452)
# 演示截图
## 首页
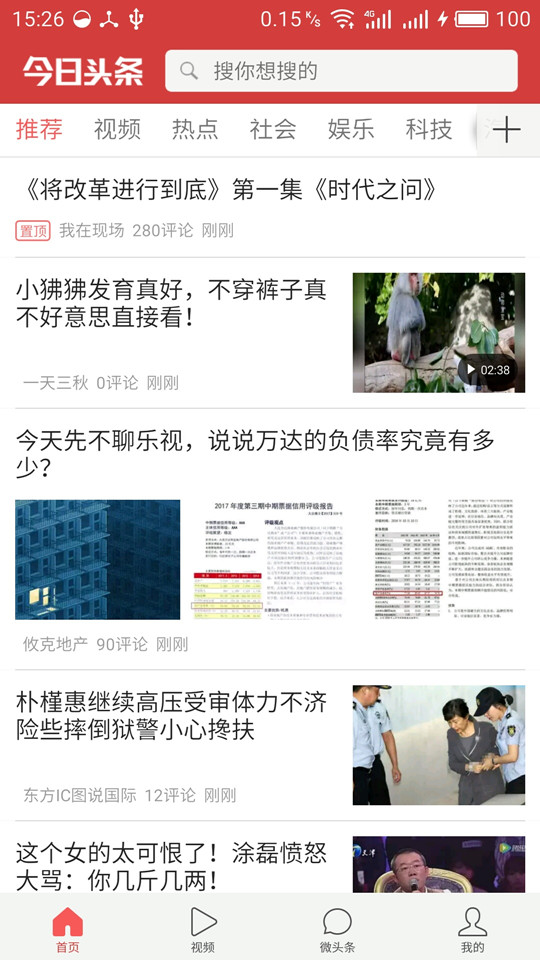
## 视频

## 微头条
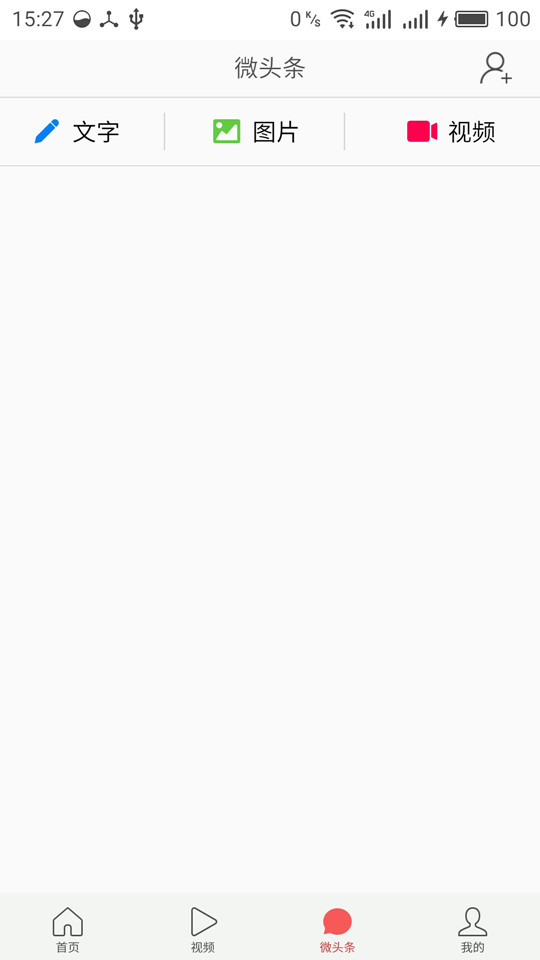
## 我的
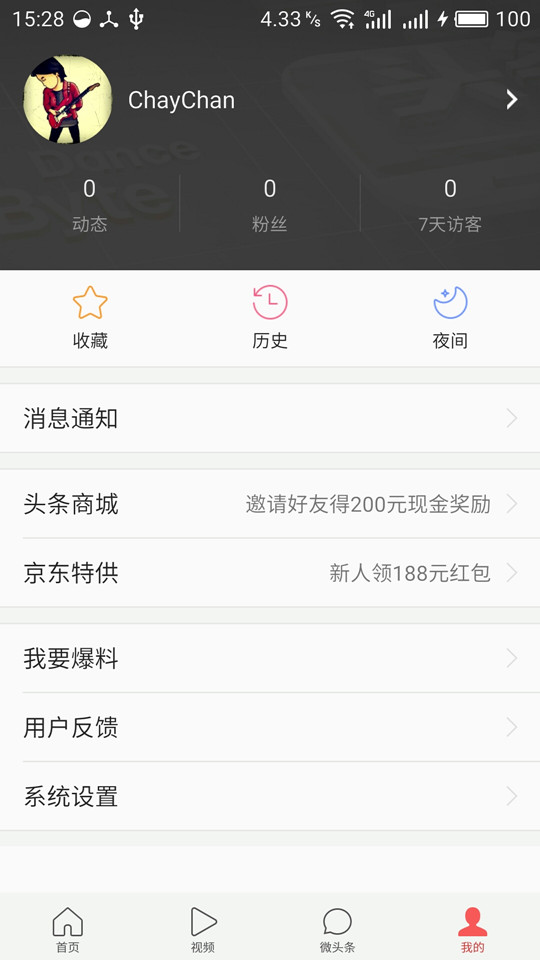
# gif图
## 新闻列表、视频列表
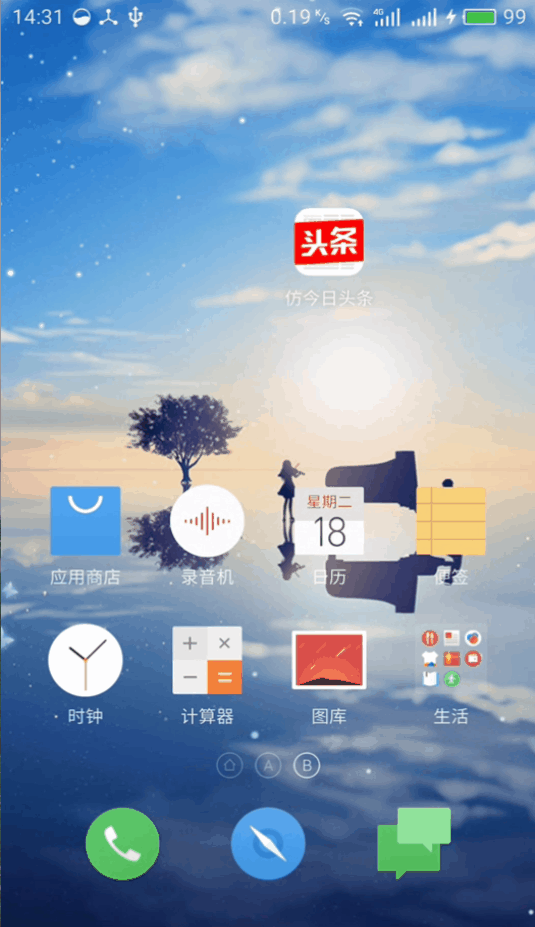
## 非视频新闻详情页面

## 查看和保存图片

## 视频播放、视频详情页面
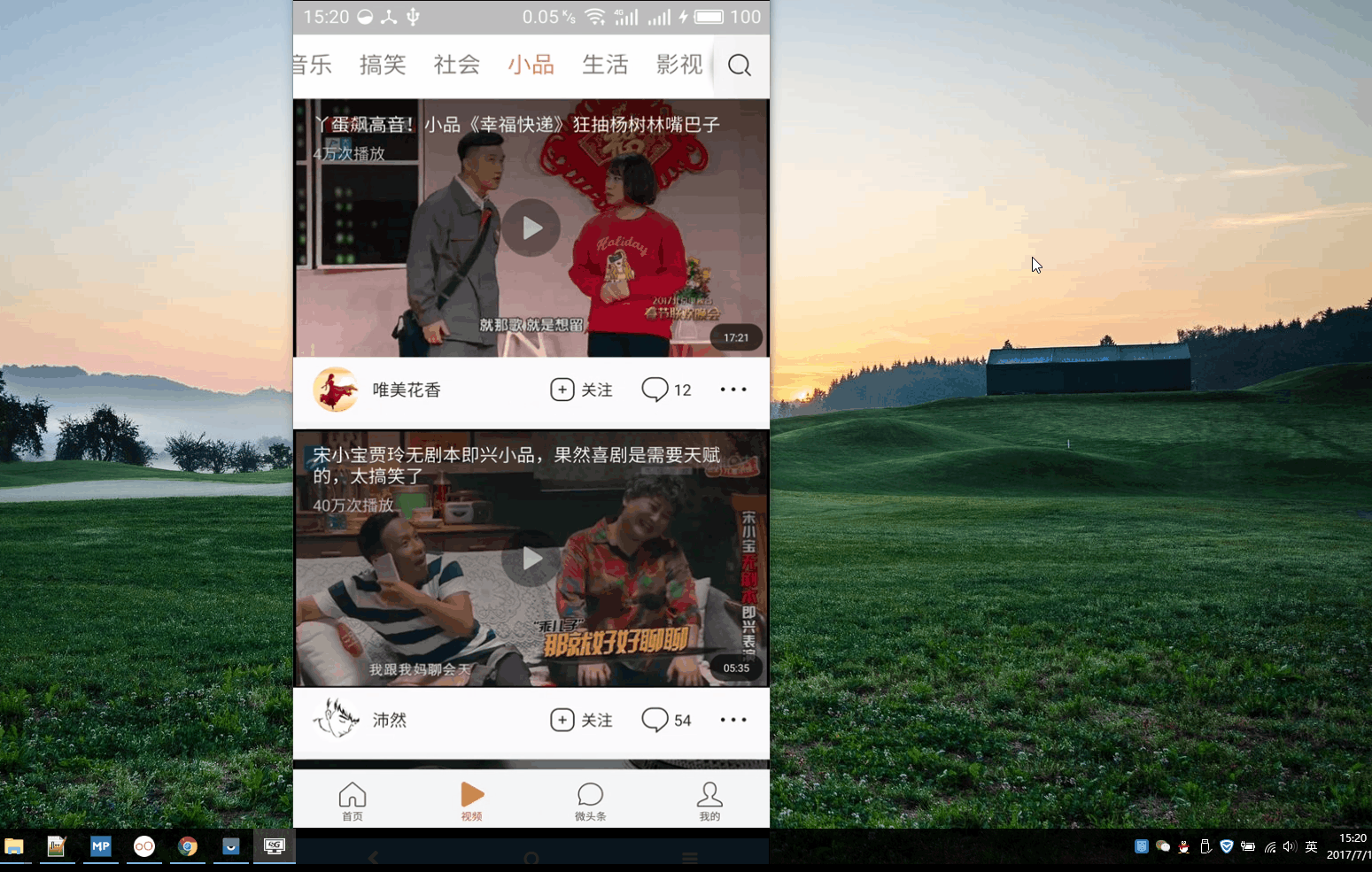
# 使用到的第三方库
* [okhttp](https://github.com/square/okhttp)
* [Retrofit](https://github.com/square/retrofit)
* [RxJava](https://github.com/ReactiveX/RxJava)
* [ButterKnife](https://github.com/JakeWharton/butterknife)
* [Gson](https://github.com/google/gson)
* [BottomBarLayout(轻量级底部导航栏)](https://github.com/chaychan/BottomBarLayout)
* [BaseRecyclerViewAdapterHelper(ReclerView万能适配器)](https://github.com/CymChad/BaseRecyclerViewAdapterHelper)
* [StateView(用于几种状态布局的切换)](https://github.com/nukc/StateView)
* [JieCaoVideoPlayer,改名 JiaoZiVideoPlayer (视频播放)](https://github.com/lipangit/JiaoZiVideoPlayer)
* [BGARefreshLayout-Android(下拉刷新)](https://github.com/bingoogolapple/BGARefreshLayout-Android)
* [Eyes(修改状态栏颜色)](https://github.com/imflyn/Eyes)
* [ColorTrackTabLayout](https://github.com/yewei02538/ColorTrackTabLayout)
* [EventBus](https://github.com/greenrobot/EventBus)
* [KLog(log管理)](https://github.com/ZhaoKaiQiang/KLog)
# 现有功能
1.获取各种频道的新闻列表,包括视频和非视频新闻;
2.查看新闻详情,包括视频和非视频新闻的详情;
3.查看新闻评论列表;
4.新闻数据本地存储,已经获取到的新闻数据保存在本地数据库中,上拉加载更多时可查看历史新闻;
5.底部页签点击下拉刷新;
6.视频列表播放中的视频不可见时停止播放。
7.查看和保存图片。
# 技术要点
1.新闻数据的抓取和分析,使用fidder抓取,具体使用可参考下面的网址:
[http://www.tuicool.com/articles/BJjQZf](http://www.tuicool.com/articles/BJjQZf)
2.新闻列表多种Item布局的展示,使用的是我封装的MultipleItemRvAdapter,基于BaseRecyclerViewAdapterHelper封装,便于多布局条目的管理:
[https://github.com/chaychan/MultipleItemRvAdapter](https://github.com/chaychan/MultipleItemRvAdapter "https://github.com/chaychan/MultipleItemRvAdapter")
3.视频源地址的解析,请查看我写的博客 [今日头条最新视频解析方法](https://blog.csdn.net/Chay_Chan/article/details/84825807)
# 声明
这个属于个人开发作品,仅做学习交流使用,如用到实际项目还需多考虑其他因素如并发等,请多多斟酌。**诸位勿传播于非技术人员,拒绝用于商业用途,数据均属于非正常渠道获取,原作公司拥有所有权利。** | 0 |
knowm/XChange | XChange is a Java library providing a streamlined API for interacting with 60+ Bitcoin and Altcoin exchanges providing a consistent interface for trading and accessing market data. | null | ## [](http://knowm.org/open-source/xchange) XChange
[](https://discord.gg/n27zjVTbDz)
[](https://github.com/knowm/XChange/actions/workflows/maven.yml)
XChange is a Java library providing a simple and consistent API for interacting with 60+ Bitcoin and other crypto currency exchanges, providing a consistent interface for trading and accessing market data.
## Important!
The world of Bitcoin changes quickly and XChange is no exception. For the latest bugfixes and features you should use the [snapshot jars](https://oss.sonatype.org/content/groups/public/org/knowm/xchange/) or build yourself from the `develop` branch. See below for more details about building with Maven. To report bugs and see what issues people are currently working on see the [issues page](https://github.com/knowm/XChange/issues).
## Description
XChange is a Java based library providing a simple and consistent API for interacting with a diverse set of crypto currency exchanges.
Basic usage is very simple: Create an `Exchange` instance, get the appropriate service, and request data. More complex usages are progressively detailed below.
## REST API
#### Public Market Data
To use public APIs which do not require authentication:
```java
Exchange bitstamp = ExchangeFactory.INSTANCE.createExchange(BitstampExchange.class);
MarketDataService marketDataService = bitstamp.getMarketDataService();
Ticker ticker = marketDataService.getTicker(CurrencyPair.BTC_USD);
System.out.println(ticker.toString());
```
#### Private Account Info
To use APIs which require authentication, create an `ExchangeSpecification` with your API credentials and pass this to `createExchange()`:
```java
ExchangeSpecification exSpec = new BitstampExchange().getDefaultExchangeSpecification();
exSpec.setUserName("34387");
exSpec.setApiKey("a4SDmpl9s6xWJS5fkKRT6yn41vXuY0AM");
exSpec.setSecretKey("sisJixU6Xd0d1yr6w02EHCb9UwYzTNuj");
Exchange bitstamp = ExchangeFactory.INSTANCE.createExchange(exSpec);
```
*Please Note:* while most exchanges use just an API key and secret key, others (such as `username` on Bitstamp or `passphrase` on Coinbase Pro) are exchange-specific. For more examples of adding the keys to the `ExchangeSpecification`, including storing them in a configuration file, see [Frequently Asked Questions](https://github.com/knowm/XChange/wiki/Frequently-Asked-Questions).
Once you have an authenticated `Exchange`, the private API services, `AccountService` and `TradeService`, can be used to access private data:
```java
// Get the account information
AccountService accountService = bitstamp.getAccountService();
AccountInfo accountInfo = accountService.getAccountInfo();
System.out.println(accountInfo.toString());
```
All exchange implementations expose the same API, but you can also directly access the underlying "raw" data from the individual exchanges if you need to.
## Websocket API
The above API is usually fully supported on all exchanges and is best used for occasional requests and polling on relatively long intervals. Many exchanges, however, heavily limit the frequency that these requests can be made, and advise instead that you use their websocket API if you need streaming or real-time data.
For a smaller number of exchanges, the websocket-based `StreamingExchange` API is also available. This uses [Reactive streams](http://reactivex.io/) to allow you to efficiently subscribe to changes relating to thousands of coin pairs without requiring large numbers of threads.
You will need to import an additional dependency for the exchange you are using (see below), then example usage is as follows:
```java
// Use StreamingExchangeFactory instead of ExchangeFactory
StreamingExchange exchange = StreamingExchangeFactory.INSTANCE.createExchange(BitstampStreamingExchange.class);
// Connect to the Exchange WebSocket API. Here we use a blocking wait.
exchange.connect().blockingAwait();
// Subscribe to live trades update.
Disposable subscription1 = exchange.getStreamingMarketDataService()
.getTrades(CurrencyPair.BTC_USD)
.subscribe(
trade -> LOG.info("Trade: {}", trade),
throwable -> LOG.error("Error in trade subscription", throwable));
// Subscribe order book data with the reference to the subscription.
Disposable subscription2 = exchange.getStreamingMarketDataService()
.getOrderBook(CurrencyPair.BTC_USD)
.subscribe(orderBook -> LOG.info("Order book: {}", orderBook));
// Wait for a while to see some results arrive
Thread.sleep(20000);
// Unsubscribe
subscription1.dispose();
subscription2.dispose();
// Disconnect from exchange (blocking again)
exchange.disconnect().blockingAwait();
```
Authentication, if supported for the exchange, works the same way as for the main API, via an `ExchangeSpecification`. For more information on what is supported, see the Wiki.
## More information
Now go ahead and [study some more examples](http://knowm.org/open-source/xchange/xchange-example-code), [download the thing](http://knowm.org/open-source/xchange/xchange-change-log/) and [provide feedback](https://github.com/knowm/XChange/issues).
More information about reactive streams can be found at the [RxJava wiki](https://github.com/ReactiveX/RxJava/wiki).
## Features
- [x] MIT license
- [x] consistent API across all implemented exchanges
- [x] active development
- [x] very minimal 3rd party dependencies
- [x] modular components
## More Info
Project Site: <http://knowm.org/open-source/xchange>
Example Code: <http://knowm.org/open-source/xchange/xchange-example-code>
Change Log: <http://knowm.org/open-source/xchange/xchange-change-log/>
Java Docs: <http://knowm.org/javadocs/xchange/index.html>
Talk to us on discord: [](https://discord.gg/n27zjVTbDz)
## Wiki
- [Home](https://github.com/knowm/XChange/wiki)
- [FAQ](https://github.com/knowm/XChange/wiki/Frequently-Asked-Questions)
- [Design Notes](https://github.com/knowm/XChange/wiki/Design-Notes)
- [Milestones](https://github.com/knowm/XChange/wiki/Milestones)
- [Exchange Support](https://github.com/knowm/XChange/wiki/Exchange-support)
- [New Implementation Best Practices](https://github.com/knowm/XChange/wiki/New-Implementation-Best-Practices)
- [Installing SSL Certificates into TrustStore](https://github.com/knowm/XChange/wiki/Installing-SSL-Certificates-into-TrustStore)
- [Getting Started with XChange for Noobies](https://github.com/knowm/XChange/wiki/Getting-Started-with-XChange-for-Noobies)
- [Code Style](https://github.com/knowm/XChange/wiki/Code-Style)
## Continuous Integration
[](https://github.com/knowm/XChange/actions/workflows/maven.yml)
## Getting Started
### Non-Maven
- [XChange Release Jars](http://search.maven.org/#search%7Cga%7C1%7Cknowm%20xchange)
- [XChange Snapshot Jars](https://oss.sonatype.org/content/groups/public/org/knowm/xchange/)
### Maven
The XChange release artifacts are hosted on Maven Central: [org.knowm.xchange](https://mvnrepository.com/artifact/org.knowm.xchange)
Add the following dependencies in your pom.xml file. You will need at least xchange-core. Add the additional dependencies for the exchange modules you are interested in (XYZ shown only for a placeholder). There is example code for all the modules in xchange-examples.
```xml
<dependency>
<groupId>org.knowm.xchange</groupId>
<artifactId>xchange-core</artifactId>
<version>5.1.1</version>
</dependency>
<dependency>
<groupId>org.knowm.xchange</groupId>
<artifactId>xchange-XYZ</artifactId>
<version>5.1.1</version>
</dependency>
```
If it is available for your exchange, you may also want to use the streaming API:
```xml
<dependency>
<groupId>org.knowm.xchange</groupId>
<artifactId>xchange-stream-XYZ</artifactId>
<version>5.1.1</version>
</dependency>
```
For snapshots, add the following repository to your pom.xml file.
```xml
<repository>
<id>sonatype-oss-snapshot</id>
<snapshots/>
<url>https://oss.sonatype.org/content/repositories/snapshots</url>
</repository>
```
The current snapshot version is:
5.1.2-SNAPSHOT
## Building with Maven
Instruction | Command
--------------------------------- | ------------------------
run unit tests | `mvn clean test`
run unit and integration tests | `mvn clean verify -DskipIntegrationTests=false`
install in local Maven repo | `mvn clean install`
create project javadocs | `mvn javadoc:aggregate`
generate dependency tree | `mvn dependency:tree`
check for dependency updates | `mvn versions:display-dependency-updates`
check for plugin updates | `mvn versions:display-plugin-updates`
code format | `mvn com.spotify.fmt:fmt-maven-plugin:format`
## Bugs
Please report any bugs or submit feature requests to [XChange's Github issue tracker](https://github.com/knowm/XChange/issues).
## Contributing
If you'd like to submit a new implementation for another exchange, please take a look at [New Implementation Best Practices](https://github.com/knowm/XChange/wiki/New-Implementation-Best-Practices) first, as there are lots of time-saving tips!
For more information such as a contributor list and a list of known projects depending on XChange, visit the [Main Project Wiki](https://github.com/knowm/XChange/wiki).
| 0 |
RedSpider1/concurrent | 这是RedSpider社区成员原创与维护的Java多线程系列文章。 | null | ## 文章地址
- [深入浅出Java多线程](http://concurrent.redspider.group/RedSpider.html)【强烈推荐!!!】
- [【GithubBook】深入浅出Java多线程](https://redspider.gitbook.io/concurrent/)
- [PDF](./book.pdf) (PDF生成比较麻烦,不会每个提交都生成,**建议看网页版本**)
## 本地运行
先安装gitbook
```bash
npm install gitbook-cli -g
```
然后安装gitbook插件
```bash
gitbook install
```
运行服务
```bash
gitbook serve .
```
然后就可以在本地 [http://localhost:4000](http://localhost:4000)访问了。
## 本书简介
笔者在读完市面上关于Java并发编程的资料后,感觉有些知识点不是很清晰,于是在RedSpider社区内展开了对Java并发编程原理的讨论。鉴于开源精神,我们决定将我们讨论之后的Java并发编程原理整理成书籍,分享给大家。
站在巨人的肩上,我们可以看得更远。本书内容的主要来源有博客、书籍、论文,对于一些已经叙述得很清晰的知识点我们直接引用在本书中;对于一些没有讲解清楚的知识点,我们加以画图或者编写Demo进行加工;而对于一些模棱两可的知识点,本书在查阅了大量资料的情况下,给出最合理的解释。
写本书的过程也是对自己研究和掌握的技术点进行整理的过程,希望本书能帮助读者快速掌握并发编程技术。
如果您或者您的单位愿意赞助本书或本社区,请发送邮件到RedSpider社区邮件组redspider@qun.mail.163.com或加微信**redspider-worker**进行洽谈。
## 勘误和支持
由于笔者的水平有限,编写时间仓促,书中难免会出现一些错误或者不准确的地方,恳请读者批评指正。如果你有更多的宝贵意见,可以在我们的github上新建issue,笔者会尽快解答,期待能够得到你的真挚反馈。github地址:https://github.com/RedSpider1/concurrent
## 公众号
欢迎关注微信公众号“编了个程”,每周会更新一篇Java方面的**原创技术文章**
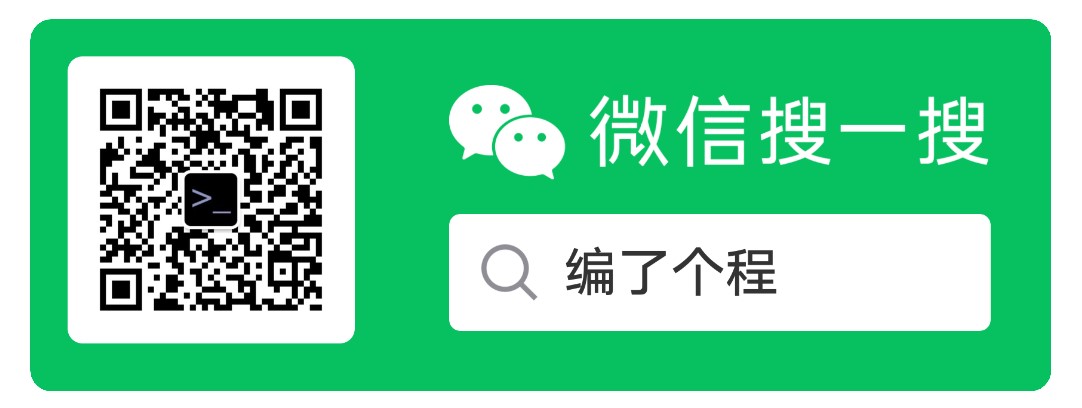 | 0 |
MiguelCatalan/MaterialSearchView | Cute library to implement SearchView in a Material Design Approach | null | # MaterialSearchView
Cute library to implement SearchView in a Material Design Approach. *Works from Android API 14 (ICS) and above*.
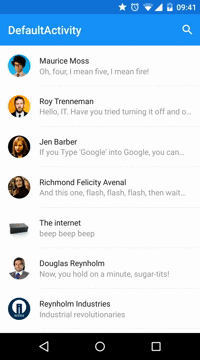 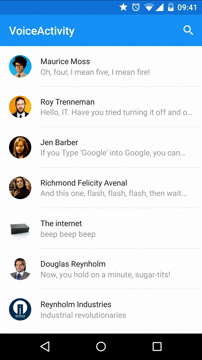
<a href="https://play.google.com/store/apps/details?id=com.miguelcatalan.materialsearchview.sample">
<img alt="Get it on Google Play"
src="https://developer.android.com/images/brand/en_generic_rgb_wo_60.png" />
</a>
#Native version
Maybe it would be useful to take a look into the new official approach
http://www.materialdoc.com/search-filter/
# Usage
**Add the dependencies to your gradle file:**
```javascript
dependencies {
compile 'com.miguelcatalan:materialsearchview:1.4.0'
}
```
**Add MaterialSearchView to your layout file along with the Toolbar** *(Add this block at the bottom of your layout, in order to display it over the rest of the view)*:
```xml
<!— Must be last for right layering display —>
<FrameLayout
android:id="@+id/toolbar_container"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="@color/theme_primary" />
<com.miguelcatalan.materialsearchview.MaterialSearchView
android:id="@+id/search_view"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</FrameLayout>
```
**Add the search item into the menu file:**
```xml
<item
android:id="@+id/action_search"
android:icon="@drawable/ic_action_action_search"
android:orderInCategory="100"
android:title="@string/abc_search_hint"
app:showAsAction="always" />
```
**Add define it in the *onCreateOptionsMenu*:**
```java
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu_main, menu);
MenuItem item = menu.findItem(R.id.action_search);
searchView.setMenuItem(item);
return true;
}
```
**Set the listeners:**
```java
MaterialSearchView searchView = (MaterialSearchView) findViewById(R.id.search_view);
searchView.setOnQueryTextListener(new MaterialSearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
//Do some magic
return false;
}
@Override
public boolean onQueryTextChange(String newText) {
//Do some magic
return false;
}
});
searchView.setOnSearchViewListener(new MaterialSearchView.SearchViewListener() {
@Override
public void onSearchViewShown() {
//Do some magic
}
@Override
public void onSearchViewClosed() {
//Do some magic
}
});
```
# Use VoiceSearch
**Allow/Disable it in the code:**
```java
searchView.setVoiceSearch(true); //or false
```
**Handle the response:**
```java
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == MaterialSearchView.REQUEST_VOICE && resultCode == RESULT_OK) {
ArrayList<String> matches = data.getStringArrayListExtra(RecognizerIntent.EXTRA_RESULTS);
if (matches != null && matches.size() > 0) {
String searchWrd = matches.get(0);
if (!TextUtils.isEmpty(searchWrd)) {
searchView.setQuery(searchWrd, false);
}
}
return;
}
super.onActivityResult(requestCode, resultCode, data);
}
```
# Add suggestions
**Define them in the resources as a *string-array*:**
```xml
<string-array name="query_suggestions">
<item>Android</item>
<item>iOS</item>
<item>SCALA</item>
<item>Ruby</item>
<item>JavaScript</item>
</string-array>
```
**Add them to the object:**
```java
searchView.setSuggestions(getResources().getStringArray(R.array.query_suggestions));
```
# Style it!
```xml
<style name="MaterialSearchViewStyle">
<!-- Background for the search bar -->
<item name="searchBackground">@color/theme_primary</item>
<!-- Change voice icon -->
<item name="searchVoiceIcon">@drawable/ic_action_voice_search_inverted</item>
<!-- Change clear text icon -->
<item name="searchCloseIcon">@drawable/ic_action_navigation_close_inverted</item>
<!-- Change up icon -->
<item name="searchBackIcon">@drawable/ic_action_navigation_arrow_back_inverted</item>
<!-- Change icon for the suggestions -->
<item name="searchSuggestionIcon">@drawable/ic_suggestion</item>
<!-- Change background for the suggestions list view -->
<item name="searchSuggestionBackground">@android:color/white</item>
<!-- Change text color for edit text. This will also be the color of the cursor -->
<item name="android:textColor">@color/theme_primary_text_inverted</item>
<!-- Change hint text color for edit text -->
<item name="android:textColorHint">@color/theme_secondary_text_inverted</item>
<!-- Hint for edit text -->
<item name="android:hint">@string/search_hint</item>
</style>
```
#Custom cursor
**Create the drawable:**
```xml
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<size android:width="2dp" />
<solid android:color="@color/theme_primary" />
</shape>
```
**And add it to the object:**
```java
searchView.setCursorDrawable(R.drawable.custom_cursor);
```
# Using AppBarLayout?
It is a little bit tricky but can be achieved using this:
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!— Irrelevant stuff —>
<android.support.v4.view.ViewPager
android:id="@+id/viewpager"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@+id/appbarlayout"
app:layout_behavior="@string/appbar_scrolling_view_behavior" />
<!— Must be last for right layering display —>
<android.support.design.widget.AppBarLayout
android:id="@+id/appbarlayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/search_layover_bg">
<FrameLayout
android:id="@+id/toolbar_container"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="@color/theme_primary" />
<com.miguelcatalan.materialsearchview.MaterialSearchView
android:id="@+id/search_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:visibility="gone" />
</FrameLayout>
<android.support.design.widget.TabLayout
android:id="@+id/tabs"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/theme_primary"
app:tabGravity="fill"
app:tabMode="fixed" />
</android.support.design.widget.AppBarLayout>
</RelativeLayout>
```
# Bonus
**Close on backpressed:**
```java
@Override
public void onBackPressed() {
if (searchView.isSearchOpen()) {
searchView.closeSearch();
} else {
super.onBackPressed();
}
}
```
# Help me
Pull requests are more than welcome, help me and others improve this awesome library.
The code is based in the Krishnakapil original concept.
# License
Copyright 2015 Miguel Catalan Bañuls
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
| 0 |
ethereum/ethereumj | DEPRECATED! Java implementation of the Ethereum yellowpaper. For JSON-RPC and other client features check Ethereum Harmony | null | # Welcome to ethereumj
[](https://gitter.im/ethereum/ethereumj?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
[](https://travis-ci.org/ethereum/ethereumj)
[](https://coveralls.io/r/ethereum/ethereumj?branch=master)
## :no_entry: Deprecated :no_entry:
This project is not supported anymore. If you have any question or would like to contribute find us on [Gitter](https://gitter.im/ethereum/ethereumj).
# About
EthereumJ is a pure-Java implementation of the Ethereum protocol. For high-level information about Ethereum and its goals, visit [ethereum.org](https://ethereum.org). The [ethereum white paper](https://github.com/ethereum/wiki/wiki/White-Paper) provides a complete conceptual overview, and the [yellow paper](http://gavwood.com/Paper.pdf) provides a formal definition of the protocol.
We keep EthereumJ as thin as possible. For [JSON-RPC](https://github.com/ethereum/wiki/wiki/JSON-RPC) support and other client features check [Ethereum Harmony](https://github.com/ether-camp/ethereum-harmony).
# Running EthereumJ
##### Adding as a dependency to your Maven project:
```
<dependency>
<groupId>org.ethereum</groupId>
<artifactId>ethereumj-core</artifactId>
<version>1.12.0-RELEASE</version>
</dependency>
```
##### or your Gradle project:
```
repositories {
mavenCentral()
jcenter()
maven { url "https://dl.bintray.com/ethereum/maven/" }
}
implementation "org.ethereum:ethereumj-core:1.9.+"
```
As a starting point for your own project take a look at https://github.com/ether-camp/ethereumj.starter
##### Building an executable JAR
```
git clone https://github.com/ethereum/ethereumj
cd ethereumj
cp ethereumj-core/src/main/resources/ethereumj.conf ethereumj-core/src/main/resources/user.conf
vim ethereumj-core/src/main/resources/user.conf # adjust user.conf to your needs
./gradlew clean fatJar
java -jar ethereumj-core/build/libs/ethereumj-core-*-all.jar
```
##### Running from command line:
```
> git clone https://github.com/ethereum/ethereumj
> cd ethereumj
> ./gradlew run [-PmainClass=<sample class>]
```
##### Optional samples to try:
```
./gradlew run -PmainClass=org.ethereum.samples.BasicSample
./gradlew run -PmainClass=org.ethereum.samples.FollowAccount
./gradlew run -PmainClass=org.ethereum.samples.PendingStateSample
./gradlew run -PmainClass=org.ethereum.samples.PriceFeedSample
./gradlew run -PmainClass=org.ethereum.samples.PrivateMinerSample
./gradlew run -PmainClass=org.ethereum.samples.TestNetSample
./gradlew run -PmainClass=org.ethereum.samples.TransactionBomb
```
##### For snapshot builds:
Please, note, snapshots are not stable and are currently in development! If you still want to try it:
- Add https://oss.jfrog.org/libs-snapshot/ as a repository to your build script
- Add a dependency on `org.ethereum:ethereumj-core:${VERSION}`, where `${VERSION}` is of the form `1.13.0-SNAPSHOT`.
Example:
<repository>
<id>jfrog-snapshots</id>
<name>oss.jfrog.org</name>
<url>https://oss.jfrog.org/libs-snapshot/</url>
<snapshots><enabled>true</enabled></snapshots>
</repository>
<!-- ... -->
<dependency>
<groupId>org.ethereum</groupId>
<artifactId>ethereumj-core</artifactId>
<version>1.13.0-SNAPSHOT</version>
</dependency>
##### Importing project to IntelliJ IDEA:
```
> git clone https://github.com/ethereum/ethereumj
> cd ethereumj
> gradlew build
```
IDEA:
* File -> New -> Project from existing sources…
* Select ethereumj/build.gradle
* Dialog “Import Project from gradle”: press “OK”
* After building run either `org.ethereum.Start`, one of `org.ethereum.samples.*` or create your own main.
# Configuring EthereumJ
For reference on all existing options, their description and defaults you may refer to the default config `ethereumj.conf` (you may find it in either the library jar or in the source tree `ethereum-core/src/main/resources`)
To override needed options you may use one of the following ways:
* put your options to the `<working dir>/config/ethereumj.conf` file
* put `user.conf` to the root of your classpath (as a resource)
* put your options to any file and supply it via `-Dethereumj.conf.file=<your config>`, accepts several configs, separated by comma applied in provided order: `-Dethereumj.conf.file=<config1>,<config2>`
* programmatically by using `SystemProperties.CONFIG.override*()`
* programmatically using by overriding Spring `SystemProperties` bean
Note that don’t need to put all the options to your custom config, just those you want to override.
# Special thanks
YourKit for providing us with their nice profiler absolutely for free.
YourKit supports open source projects with its full-featured Java Profiler.
YourKit, LLC is the creator of <a href="https://www.yourkit.com/java/profiler/">YourKit Java Profiler</a>
and <a href="https://www.yourkit.com/.net/profiler/">YourKit .NET Profiler</a>,
innovative and intelligent tools for profiling Java and .NET applications.

# Contact
Chat with us via [Gitter](https://gitter.im/ethereum/ethereumj)
# License
ethereumj is released under the [LGPL-V3 license](LICENSE).
| 0 |
vsch/flexmark-java | CommonMark/Markdown Java parser with source level AST. CommonMark 0.28, emulation of: pegdown, kramdown, markdown.pl, MultiMarkdown. With HTML to MD, MD to PDF, MD to DOCX conversion modules. | commonmark html-to-markdown java markdown markdown-conversion markdown-flavors markdown-parser markdown-processor markdown-to-html markdown-to-pdf pegdown |  flexmark-java
=========================================================================
**flexmark-java** is a Java implementation of **[CommonMark (spec 0.28)]** parser using the
blocks first, inlines after Markdown parsing architecture.
Its strengths are speed, flexibility, Markdown source element based AST with details of the
source position down to individual characters of lexemes that make up the element and
extensibility.
The API allows granular control of the parsing process and is optimized for parsing with a large
number of installed extensions. The parser and extensions come with plenty of options for parser
behavior and HTML rendering variations. The end goal is to have the parser and renderer be able
to mimic other parsers with great degree of accuracy. This is now partially complete with the
implementation of [Markdown Processor Emulation](#markdown-processor-emulation)
Motivation for this project was the need to replace [pegdown] parser in my [Markdown Navigator]
plugin for JetBrains IDEs. [pegdown] has a great feature set but its speed in general is less
than ideal and for pathological input either hangs or practically hangs during parsing.
:warning: **Version 0.60.0** has breaking changes due to re-organization, renaming, clean up and
optimization of implementation classes. Changes are detailed in
[Version-0.60.0-Changes](../../wiki/Version-0.60.0-Changes).
### latest [](https://search.maven.org/search?q=g:com.vladsch.flexmark)<!-- @IGNORE PREVIOUS: link --> [](https://www.javadoc.io/doc/com.vladsch.flexmark/flexmark)
### Requirements
* For Versions 0.62.2 or below, Java 8 or above, Java 9+ compatible. For Versions 0.64.0 or
above, Java 11 or above.
* The project is on Maven: `com.vladsch.flexmark`
* The core has no dependencies other than `org.jetbrains:annotations:24.0.1`. For extensions, see
extension description below.
The API is still evolving to accommodate new extensions and functionality.
### Quick Start
For Maven, add `flexmark-all` as a dependency which includes core and all modules to the
following sample:
```xml
<dependency>
<groupId>com.vladsch.flexmark</groupId>
<artifactId>flexmark-all</artifactId>
<version>0.64.8</version>
</dependency>
```
Source:
[BasicSample.java](flexmark-java-samples/src/com/vladsch/flexmark/java/samples/BasicSample.java)
```java
package com.vladsch.flexmark.samples;
import com.vladsch.flexmark.util.ast.Node;
import com.vladsch.flexmark.html.HtmlRenderer;
import com.vladsch.flexmark.parser.Parser;
import com.vladsch.flexmark.util.data.MutableDataSet;
public class BasicSample {
public static void main(String[] args) {
MutableDataSet options = new MutableDataSet();
// uncomment to set optional extensions
//options.set(Parser.EXTENSIONS, Arrays.asList(TablesExtension.create(), StrikethroughExtension.create()));
// uncomment to convert soft-breaks to hard breaks
//options.set(HtmlRenderer.SOFT_BREAK, "<br />\n");
Parser parser = Parser.builder(options).build();
HtmlRenderer renderer = HtmlRenderer.builder(options).build();
// You can re-use parser and renderer instances
Node document = parser.parse("This is *Sparta*");
String html = renderer.render(document); // "<p>This is <em>Sparta</em></p>\n"
System.out.println(html);
}
}
```
#### Building via Gradle
```shell
implementation 'com.vladsch.flexmark:flexmark-all:0.64.8'
```
#### Building with Android Studio
Additional settings due to duplicate files:
```groovy
packagingOptions {
exclude 'META-INF/LICENSE-LGPL-2.1.txt'
exclude 'META-INF/LICENSE-LGPL-3.txt'
exclude 'META-INF/LICENSE-W3C-TEST'
exclude 'META-INF/DEPENDENCIES'
}
```
More information can be found in the documentation:
[Wiki Home](../../wiki) [Usage Examples](../../wiki/Usage)
[Extension Details](../../wiki/Extensions)
[Writing Extensions](../../wiki/Writing-Extensions)
### Pegdown Migration Helper
`PegdownOptionsAdapter` class converts pegdown `Extensions.*` flags to flexmark options and
extensions list. Pegdown `Extensions.java` is included for convenience and new options not found
in pegdown 1.6.0. These are located in `flexmark-profile-pegdown` module but you can grab the
source from this repo: [PegdownOptionsAdapter.java], [Extensions.java] and make your own
version, modified to your project's needs.
You can pass your extension flags to static `PegdownOptionsAdapter.flexmarkOptions(int)` or you
can instantiate `PegdownOptionsAdapter` and use convenience methods to set, add and remove
extension flags. `PegdownOptionsAdapter.getFlexmarkOptions()` will return a fresh copy of
`DataHolder` every time with the options reflecting pegdown extension flags.
```java
import com.vladsch.flexmark.html.HtmlRenderer;
import com.vladsch.flexmark.parser.Parser;
import com.vladsch.flexmark.profile.pegdown.Extensions;
import com.vladsch.flexmark.profile.pegdown.PegdownOptionsAdapter;
import com.vladsch.flexmark.util.data.DataHolder;
public class PegdownOptions {
final private static DataHolder OPTIONS = PegdownOptionsAdapter.flexmarkOptions(
Extensions.ALL
);
static final Parser PARSER = Parser.builder(OPTIONS).build();
static final HtmlRenderer RENDERER = HtmlRenderer.builder(OPTIONS).build();
// use the PARSER to parse and RENDERER to render with pegdown compatibility
}
```
Default flexmark-java pegdown emulation uses less strict HTML block parsing which interrupts an
HTML block on a blank line. Pegdown only interrupts an HTML block on a blank line if all tags in
the HTML block are closed.
To get closer to original pegdown HTML block parsing behavior use the method which takes a
`boolean strictHtml` argument:
```java
import com.vladsch.flexmark.html.HtmlRenderer;
import com.vladsch.flexmark.parser.Parser;
import com.vladsch.flexmark.profile.pegdown.Extensions;
import com.vladsch.flexmark.profile.pegdown.PegdownOptionsAdapter;
import com.vladsch.flexmark.util.data.DataHolder;
public class PegdownOptions {
final private static DataHolder OPTIONS = PegdownOptionsAdapter.flexmarkOptions(true,
Extensions.ALL
);
static final Parser PARSER = Parser.builder(OPTIONS).build();
static final HtmlRenderer RENDERER = HtmlRenderer.builder(OPTIONS).build();
// use the PARSER to parse and RENDERER to render with pegdown compatibility
}
```
A sample with a [custom link resolver] is also available, which includes link resolver for
changing URLs or attributes of links and a custom node renderer if you need to override the
generated link HTML.
:information_source: [flexmark-java] has many more extensions and configuration options than
[pegdown] in addition to extensions available in pegdown 1.6.0.
[Available Extensions via PegdownOptionsAdapter](../../wiki/Pegdown-Migration#available-extensions-via-pegdownoptionsadapter)
### Latest Additions and Changes
* Major reorganization and code cleanup of implementation in version 0.60.0, see
[Version-0.60.0-Changes](../../wiki/Version-0.60.0-Changes) thanks to great work by
[Alex Karezin](mailto:javadiagrams@gmail.com) you can get an overview of module dependencies
with ability to drill down to packages and classes.
* [Merge API](../../wiki/Markdown-Merge-API) to merge multiple markdown documents into a single
document.
* Docx Renderer Extension:
[Limited Attributes Node Handling](../../wiki/Docx-Renderer-Extension#limited-attributes-node-handling)
* Extensible HTML to Markdown Converter module:
[flexmark-html2md-converter](flexmark-html2md-converter). Sample:
[HtmlToMarkdownCustomizedSample.java]
* Java9+ module compatibility
* Compound Enumerated References
[Enumerated References Extension](../../wiki/Enumerated-References-Extension) for creating
legal numbering for elements and headings.
* [Macros Extension](../../wiki/Macros-Extension) to allow arbitrary markdown content to be
inserted as block or inline elements, allowing block elements to be used where only inline
elements are allowed by syntax.
* [GitLab Flavoured Markdown](../../wiki/Extensions#gitlab-flavoured-markdown) for parsing and
rendering GitLab markdown extensions.
* OSGi module courtesy Dan Klco (GitHub [@klcodanr](https://github.com/klcodanr))
* [Media Tags](../../wiki/Extensions#media-tags) Media link transformer extension courtesy
Cornelia Schultz (GitHub [@CorneliaXaos](https://github.com/CorneliaXaos)) transforms links
using custom prefixes to Audio, Embed, Picture, and Video HTML5 tags.
* [Translation Helper API](../../wiki/Translation-Helper-API) to make translating markdown
documents easier.
* [Admonition](../../wiki/Extensions#admonition) To create block-styled side content. For
complete documentation please see the [Admonition Extension, Material for MkDocs]
documentation.
* [Enumerated Reference](../../wiki/Extensions#enumerated-reference) to create enumerated
references for figures, tables and other markdown elements.
* [Attributes](../../wiki/Extensions#attributes) to parse attributes of the form `{name
name=value name='value' name="value" #id .class-name}` attributes.
* [YouTube Embedded Link Transformer](../../wiki/Extensions#youtube-embedded-link-transformer)
thanks to Vyacheslav N. Boyko (GitHub @bvn13) transforms simple links to youtube videos to
embedded video iframe HTML.
* [Docx Converter](../../wiki/Extensions#docx-converter) using the [docx4j] library. How to use:
[DocxConverter Sample], how to customize:
[Customizing Docx Rendering](../../wiki/Customizing-Docx-Rendering)
Development of this module was sponsored by
[Johner Institut GmbH](https://www.johner-institut.de).
* Update library to be [CommonMark (spec 0.28)] compliant and add
`ParserEmulationProfile.COMMONMARK_0_27` and `ParserEmulationProfile.COMMONMARK_0_28` to allow
selecting a specific spec version options.
* Custom node rendering API with ability to invoke standard rendering for an overridden node,
allowing custom node renders that only handle special cases and let the rest be rendered as
usual. [custom link resolver]
* [Gfm-Issues](../../wiki/Extensions#gfm-issues) and
[Gfm-Users](../../wiki/Extensions#gfm-users) extensions for parsing and rendering `#123` and
`@user-name` respectively.
* Deep HTML block parsing option for better handling of raw text tags that come after other tags
and for [pegdown] HTML block parsing compatibility.
* `flexmark-all` module that includes: core, all extensions, formatter, JIRA and YouTrack
converters, pegdown profile module and HTML to Markdown conversion.
* [PDF Output Module](../../wiki/Extensions#pdf-output-module)
[PDF output](../../wiki/Usage#pdf-output) using [Open HTML To PDF]
* [Typographic](../../wiki/Extensions#typographic) implemented
* [XWiki Macro Extension](../../wiki/Extensions#xwiki-macro-extension)
* [Jekyll Tags](../../wiki/Extensions#jekyll-tags)
* [Html To Markdown](../../wiki/Extensions#html-to-markdown)
* [Maven Markdown Page Generator Plugin](https://github.com/vsch/markdown-page-generator-plugin)
* [Markdown Formatter](../../wiki/Markdown-Formatter) module to output AST as markdown with
formatting options.
* [Tables](../../wiki/Extensions#tables) for [Markdown Formatter](../../wiki/Markdown-Formatter)
with column width and alignment of markdown tables:
<table>
<thead> <tr><th>Input</th> <th>Output</th> </tr> </thead>
<tr><td>
<pre><code class="language-markdown">day|time|spent
:---|:---:|--:
nov. 2. tue|10:00|4h 40m
nov. 3. thu|11:00|4h
nov. 7. mon|10:20|4h 20m
total:|| 13h</code></pre>
</td><td>
<pre><code class="language-markdown">| day | time | spent |
|:------------|:-----:|--------:|
| nov. 2. tue | 10:00 | 4h 40m |
| nov. 3. thu | 11:00 | 4h |
| nov. 7. mon | 10:20 | 4h 20m |
| total: || 13h |</code></pre>
</td></tr>
</table>
### Releases, Bug Fixes, Enhancements and Support
I use flexmark-java as the parser for [Markdown Navigator] plugin for JetBrains IDEs. I tend to
use the latest, unreleased version to fix bugs or get improvements. So if you find a bug that is
a show stopper for your project or see a bug in [github issues page] marked `fixed for next
release` that is affecting your project then please let me know and I may be able to promptly
make a new release to address your issue. Otherwise, I will let bug fixes and enhancements
accumulate thinking no one is affected by what is already fixed.
#### Extension points in the API are many and numerous
There are many extension options in the API with their intended use. A good soft-start is the
[`flexmark-java-samples`](flexmark-java-samples) module which has simple samples for asked for
extensions. The next best place is the source of an existing extension that has similar syntax
to what you want to add.
If your extension lines up with the right API, the task is usually very short and sweet. If your
extension uses the API in an unintended fashion or does not follow expected housekeeping
protocols, you may find it an uphill battle with a rat's nest of if/else condition handling and
fixing one bug only leading to creating another one.
Generally, if it takes more than a few dozen lines to add a simple extension, then either you
are going about it wrong or the API is missing an extension point. If you look at all the
implemented extensions you will see that most are a few lines of code other than boiler plate
dictated by the API. That is the goal for this library: provide an extensible core that makes
writing extensions a breeze.
The larger extensions are `flexmark-ext-tables` and `flexmark-ext-spec-example`, the meat of
both is around 200 lines of code. You can use them as a guide post for size estimating your
extension.
My own experience adding extensions shows that sometimes a new type of extension is best
addressed with an API enhancement to make its implementation seamless, or by fixing a bug that
was not visible before the extension stressed the API in just the right way. Your intended
extension may just be the one requiring such an approach.
#### Don't hesitate to open an issue if you can't find the answer
The takeaway is: if you want to implement an extension or a feature please don't hesitate to
open an issue and I will give you pointers on the best way to go about it. It may save you a lot
of time by letting me improve the API to address your extension's needs before you put a lot of
fruitless effort into it.
I do ask that you realize that I am chief cook and bottle washer on this project, without an
iota of Vulcan Mind Melding skills. I do ask that you describe what you want to implement
because I can't read your mind. Please do some reconnaissance background work around the source
code and documentation because I cannot transfer what I know to you, without your willing
effort.
#### Consulting is available
If you have a commercial application and don't want to write the extension(s) yourself or want
to reduce the time and effort of implementing extensions and integrating flexmark-java, feel
free to contact me. I am available on a consulting/contracting basis.
### Markdown Processor Emulation
Despite its name, commonmark is neither a superset nor a subset of other markdown flavors.
Rather, it proposes a standard, unambiguous syntax specification for the original, "core"
Markdown, thus effectively introducing yet another flavor. While flexmark is by default
commonmark compliant, its parser can be tweaked in various ways. The sets of tweaks required to
emulate the most commonly used markdown parsers around are available in flexmark as
`ParserEmulationProfiles`.
As the name `ParserEmulationProfile` implies, it's only the parser that is adjusted to the
specific markdown flavor. Applying the profile does not add features beyond those available in
commonmark. If you want to use flexmark to fully emulate another markdown processor's behavior,
you have to adjust the parser and configure the flexmark extensions that provide the additional
features available in the parser that you want to emulate.
A rewrite of the list parser to better control emulation of other markdown processors as per
[Markdown Processors Emulation](MarkdownProcessorsEmulation.md) is complete. Addition of
processor presets to emulate specific markdown processing behaviour of these parsers is on a
short to do list.
Some emulation families do a better better job of emulating their target than others. Most of
the effort was directed at emulating how these processors parse standard Markdown and list
related parsing specifically. For processors that extend original Markdown, you will need to add
those extensions that are already implemented in flexmark-java to the Parser/Renderer builder
options.
Extensions will be modified to include their own presets for specific processor emulation, if
that processor has an equivalent extension implemented.
If you find a discrepancy please open an issue so it can be addressed.
Major processor families are implemented and some family members also:
* [ ] [Jekyll]
* [CommonMark] for latest implemented spec, currently [CommonMark (spec 0.28)]
* [ ] [League/CommonMark]
* [CommonMark (spec 0.27)] for specific version compatibility
* [CommonMark (spec 0.28)] for specific version compatibility
* [GitHub] Comments
* [Markdown.pl][Markdown]
* [ ] [Php Markdown Extra]
* [GitHub] Docs (old GitHub markdown parser)
* [Kramdown]
* FixedIndent
* [MultiMarkdown]
* [Pegdown]
:information_source: profiles to encapsulate configuration details for variants within the
family were added in 0.11.0:
* CommonMark (default for family): `ParserEmulationProfile.COMMONMARK`
* FixedIndent (default for family): `ParserEmulationProfile.FIXED_INDENT`
* GitHub Comments (just CommonMark): `ParserEmulationProfile.COMMONMARK`
* Old GitHub Docs: `ParserEmulationProfile.GITHUB_DOC`
* Kramdown (default for family): `ParserEmulationProfile.KRAMDOWN`
* Markdown.pl (default for family): `ParserEmulationProfile.MARKDOWN`
* MultiMarkdown: `ParserEmulationProfile.MULTI_MARKDOWN`
* Pegdown, with pegdown extensions use `PegdownOptionsAdapter` in `flexmark-profile-pegdown`
* Pegdown, without pegdown extensions `ParserEmulationProfile.PEGDOWN`
* Pegdown HTML block parsing rules, without pegdown extensions
`ParserEmulationProfile.PEGDOWN_STRICT`
### History and Motivation
**flexmark-java** is a fork of [commonmark-java] project, modified to generate an AST which
reflects all the elements in the original source, full source position tracking for all elements
in the AST and easier JetBrains Open API PsiTree generation.
The API was changed to allow more granular control of the parsing process and optimized for
parsing with a large number of installed extensions. The parser and extensions come with many
tweaking options for parser behavior and HTML rendering variations. The end goal is to have the
parser and renderer be able to mimic other parsers with great degree of accuracy.
Motivation for this was the need to replace [pegdown] parser in [Markdown Navigator] plugin.
[pegdown] has a great feature set but its speed in general is less than ideal and for
pathological input either hangs or practically hangs during parsing.
[commonmark-java] has an excellent parsing architecture that is easy to understand and extend.
The goal was to ensure that adding source position tracking in the AST would not change the ease
of parsing and generating the AST more than absolutely necessary.
Reasons for choosing [commonmark-java] as the parser are: speed, ease of understanding, ease of
extending and speed. Now that I have reworked the core and added a few extensions I am extremely
satisfied with my choice.
Another goal was to improve the ability of extensions to modify parser behavior so that any
dialect of markdown could be implemented through the extension mechanism. An extensible options
API was added to allow setting of all options in one place. Parser, renderer and extensions use
these options for configuration, including disabling some core block parsers.
This is a work in progress with many API changes. No attempt is made to keep backward API
compatibility to the original project and until the feature set is mostly complete, not even to
earlier versions of this project.
### Feature Comparison
| Feature | flexmark-java | commonmark-java | pegdown |
|:---------------------------------------------------------------------------------|:-----------------------------------------------------------------|:------------------------------------------------------------------|:---------------------------------------------------------------------|
| Relative parse time (less is better) | :heavy_check_mark: 1x [(1)](#1) | :heavy_check_mark: 0.6x to 0.7x [(2)](#2) | :x: 25x average, 20,000x to ∞ for pathological input [(3)](#3) |
| All source elements in the AST | :heavy_check_mark: | :x: | :heavy_check_mark: |
| AST elements with source position | :heavy_check_mark: | :heavy_check_mark: | :heavy_check_mark: with some errors and idiosyncrasies |
| AST can be easily manipulated | :heavy_check_mark: AST post processing is an extension mechanism | :heavy_check_mark: AST post processing is an extension mechanism | :x: not an option. No node's parent information, children as List<>. |
| AST elements have detailed source position for all parts | :heavy_check_mark: | :x: | :x: only node start/end |
| Can disable core parsing features | :heavy_check_mark: | :x: | :x: |
| Core parser implemented via the extension API | :heavy_check_mark: | :x: `instanceOf` tests for specific block parser and node classes | :x: core exposes few extension points |
| Easy to understand and modify parser implementation | :heavy_check_mark: | :heavy_check_mark: | :x: one PEG parser with complex interactions [(3)](#3) |
| Parsing of block elements is independent from each other | :heavy_check_mark: | :heavy_check_mark: | :x: everything in one PEG grammar |
| Uniform configuration across: parser, renderer and all extensions | :heavy_check_mark: | :x: none beyond extension list | :x: `int` bit flags for core, none for extensions |
| Parsing performance optimized for use with extensions | :heavy_check_mark: | :x: parsing performance for core, extensions do what they can | :x: performance is not a feature |
| Feature rich with many configuration options and extensions out of the box | :heavy_check_mark: | :x: limited extensions, no options | :heavy_check_mark: |
| Dependency definitions for processors to guarantee the right order of processing | :heavy_check_mark: | :x: order specified by extension list ordering, error prone | :x: not applicable, core defines where extension processing is added |
<!--
| | :x: | :x: | :x: |
| | :x: | :x: | :x: |
| | :x: | :x: | :x: |
| | :x: | :x: | :x: |
| | :x: | :x: | :x: |
|--|:----|:----|:----|
| | :x: | :x: | :x: |
-->
###### (1)
flexmark-java pathological input of 100,000 `[` parses in 68ms, 100,000 `]` in 57ms, 100,000
nested `[` `]` parse in 55ms
###### (2)
commonmark-java pathological input of 100,000 `[` parses in 30ms, 100,000 `]` in 30ms, 100,000
nested `[` `]` parse in 43ms
###### (3)
pegdown pathological input of 17 `[` parses in 650ms, 18 `[` in 1300ms
Progress
--------
* Parser options, items marked as a task item are to be implemented the rest are complete:
* Typographic
* Quotes
* Smarts
* GitHub Extensions
* Fenced code blocks
* Anchor links for headers with auto id generation
* Table Spans option to be implemented for tables extension
* Wiki Links with GitHub and Creole syntax
* Emoji Shortcuts with use GitHub emoji URL option
* GitHub Syntax
* Strikethrough
* Task Lists
* No Atx Header Space
* No Header indents
* Hard Wraps (achieved with SOFT_BREAK option changed to `"<br />"`)
* Relaxed HR Rules Option
* Wiki links
* Publishing
* Abbreviations
* Footnotes
* Definitions
* Table of Contents
* Suppress
* inline HTML: all, non-comments, comments
* HTML blocks: all, non-comments, comments
* Processor Extensions
* Jekyll front matter
* Jekyll tag elements, with support for `{% include file %}`,
[Include Markdown and HTML File Content]
* GitBook link URL encoding. Not applicable
* HTML comment nodes: Block and Inline
* Multi-line Image URLs
* Spec Example Element
* Commonmark Syntax suppression
* Manual loose lists
* Numbered lists always start with 1.
* Fixed list item indent, items must be indented by at least 4 spaces
* Relaxed list start option, allow lists to start when not preceded by a blank line.
I am very pleased with the decision to switch to [commonmark-java] based parser for my own
projects. Even though I had to do major surgery on its innards to get full source position
tracking and AST that matches source elements, it is a pleasure to work with and is now a
pleasure to extend. If you don't need source level element AST or the rest of what flexmark-java
added and [CommonMark] is your target markdown parser then I encourage you to use
[commonmark-java] as it is an excellent choice for your needs and its performance does not
suffer for the overhead of features that you will not use.
Benchmarks
----------
Latest, Jan 28, 2017 flexmark-java 0.13.1, intellij-markdown from CE EAP 2017, commonmark-java
0.8.0:
| File | commonmark-java | flexmark-java | intellij-markdown | pegdown |
|:-----------------|----------------:|--------------:|------------------:|----------:|
| README-SLOW | 0.420ms | 0.812ms | 2.027ms | 15.483ms |
| VERSION | 0.743ms | 1.425ms | 4.057ms | 42.936ms |
| commonMarkSpec | 31.025ms | 44.465ms | 600.654ms | 575.131ms |
| markdown_example | 8.490ms | 10.502ms | 223.593ms | 983.640ms |
| spec | 4.719ms | 6.249ms | 35.883ms | 307.176ms |
| table | 0.229ms | 0.623ms | 0.800ms | 3.642ms |
| table-format | 1.385ms | 2.881ms | 4.150ms | 23.592ms |
| wrap | 3.804ms | 4.589ms | 16.609ms | 86.383ms |
Ratios of above:
| File | commonmark-java | flexmark-java | intellij-markdown | pegdown |
|:-----------------|----------------:|--------------:|------------------:|----------:|
| README-SLOW | 1.00 | 1.93 | 4.83 | 36.88 |
| VERSION | 1.00 | 1.92 | 5.46 | 57.78 |
| commonMarkSpec | 1.00 | 1.43 | 19.36 | 18.54 |
| markdown_example | 1.00 | 1.24 | 26.34 | 115.86 |
| spec | 1.00 | 1.32 | 7.60 | 65.09 |
| table | 1.00 | 2.72 | 3.49 | 15.90 |
| table-format | 1.00 | 2.08 | 3.00 | 17.03 |
| wrap | 1.00 | 1.21 | 4.37 | 22.71 |
| **overall** | **1.00** | **1.41** | **17.47** | **40.11** |
| File | commonmark-java | flexmark-java | intellij-markdown | pegdown |
|:-----------------|----------------:|--------------:|------------------:|----------:|
| README-SLOW | 0.52 | 1.00 | 2.50 | 19.07 |
| VERSION | 0.52 | 1.00 | 2.85 | 30.12 |
| commonMarkSpec | 0.70 | 1.00 | 13.51 | 12.93 |
| markdown_example | 0.81 | 1.00 | 21.29 | 93.66 |
| spec | 0.76 | 1.00 | 5.74 | 49.15 |
| table | 0.37 | 1.00 | 1.28 | 5.85 |
| table-format | 0.48 | 1.00 | 1.44 | 8.19 |
| wrap | 0.83 | 1.00 | 3.62 | 18.83 |
| **overall** | **0.71** | **1.00** | **12.41** | **28.48** |
---
Because these two files represent the pathological input for pegdown, I no longer run them as
part of the benchmark to prevent skewing of the results. The results are here for posterity.
| File | commonmark-java | flexmark-java | intellij-markdown | pegdown |
|:--------------|----------------:|--------------:|------------------:|-----------:|
| hang-pegdown | 0.082ms | 0.326ms | 0.342ms | 659.138ms |
| hang-pegdown2 | 0.048ms | 0.235ms | 0.198ms | 1312.944ms |
Ratios of above:
| File | commonmark-java | flexmark-java | intellij-markdown | pegdown |
|:--------------|----------------:|--------------:|------------------:|-------------:|
| hang-pegdown | 1.00 | 3.98 | 4.17 | 8048.38 |
| hang-pegdown2 | 1.00 | 4.86 | 4.10 | 27207.32 |
| **overall** | **1.00** | **4.30** | **4.15** | **15151.91** |
| File | commonmark-java | flexmark-java | intellij-markdown | pegdown |
|:--------------|----------------:|--------------:|------------------:|------------:|
| hang-pegdown | 0.25 | 1.00 | 1.05 | 2024.27 |
| hang-pegdown2 | 0.21 | 1.00 | 0.84 | 5594.73 |
| **overall** | **0.23** | **1.00** | **0.96** | **3519.73** |
* [VERSION.md] is the version log file I use for Markdown Navigator
* [commonMarkSpec.md] is a 33k line file used in [intellij-markdown] test suite for performance
evaluation.
* [spec.txt] commonmark spec markdown file in the [commonmark-java] project
* [hang-pegdown.md] is a file containing a single line of 17 characters `[[[[[[[[[[[[[[[[[`
which causes pegdown to go into a hyper-exponential parse time.
* [hang-pegdown2.md] a file containing a single line of 18 characters `[[[[[[[[[[[[[[[[[[` which
causes pegdown to go into a hyper-exponential parse time.
* [wrap.md] is a file I was using to test wrap on typing performance only to discover that it
has nothing to do with the wrap on typing code when 0.1 seconds is taken by pegdown to parse
the file. In the plugin the parsing may happen more than once: syntax highlighter pass, psi
tree building pass, external annotator.
* markdown_example.md a file with 10,000+ lines containing 500kB+ of text.
Contributing
------------
Pull requests, issues and comments welcome :smile:. For pull requests:
* Add tests for new features and bug fixes, preferably in the
[ast_spec.md](flexmark-core-test/src/test/resources/ast_spec.md) format
* Follow the existing style to make merging easier, as much as possible: 4 space indent,
trailing spaces trimmed.
* * *
License
-------
Copyright (c) 2015-2016 Atlassian and others.
Copyright (c) 2016-2023, Vladimir Schneider,
BSD (2-clause) licensed, see [LICENSE.txt] file.
[Admonition Extension, Material for MkDocs]: https://squidfunk.github.io/mkdocs-material/reference/admonitions/
[CommonMark]: https://commonmark.org
[CommonMark (spec 0.27)]: https://spec.commonmark.org/0.27
[CommonMark (spec 0.28)]: https://spec.commonmark.org/0.28
[DocxConverter Sample]: flexmark-java-samples/src/com/vladsch/flexmark/java/samples/DocxConverterCommonMark.java
[Extensions.java]: flexmark-profile-pegdown/src/main/java/com/vladsch/flexmark/profile/pegdown/Extensions.java
[GitHub]: https://github.com/vsch/laravel-translation-manager
[GitHub Issues page]: ../../issues
[HtmlToMarkdownCustomizedSample.java]: flexmark-java-samples/src/com/vladsch/flexmark/java/samples/HtmlToMarkdownCustomizedSample.java
[Include Markdown and HTML File Content]: ../../wiki/Usage#include-markdown-and-html-file-content
[Jekyll]: https://jekyllrb.com
[Kramdown]: https://kramdown.gettalong.org
[LICENSE.txt]: LICENSE.txt
[League/CommonMark]: https://github.com/thephpleague/commonmark
[Markdown]: https://daringfireball.net/projects/markdown/
[Markdown Navigator]: https://github.com/vsch/idea-multimarkdown
[MultiMarkdown]: https://fletcherpenney.net/multimarkdown
[Open HTML To PDF]: https://github.com/danfickle/openhtmltopdf
[PHP Markdown Extra]: https://michelf.ca/projects/php-markdown/extra/#abbr
[PegdownOptionsAdapter.java]: flexmark-profile-pegdown/src/main/java/com/vladsch/flexmark/profile/pegdown/PegdownOptionsAdapter.java
[VERSION.md]: https://github.com/vsch/idea-multimarkdown/blob/master/test-data/performance/VERSION.md
[commonmark-java]: https://github.com/atlassian/commonmark-java
[commonMarkSpec.md]: https://github.com/vsch/idea-multimarkdown/blob/master/test-data/performance/commonMarkSpec.md
[custom link resolver]: flexmark-java-samples/src/com/vladsch/flexmark/java/samples/PegdownCustomLinkResolverOptions.java
[docx4j]: https://www.docx4java.org/trac/docx4j
[flexmark-java]: https://github.com/vsch/flexmark-java
[hang-pegdown.md]: https://github.com/vsch/idea-multimarkdown/blob/master/test-data/performance/hang-pegdown.md
[hang-pegdown2.md]: https://github.com/vsch/idea-multimarkdown/blob/master/test-data/performance/hang-pegdown2.md
[intellij-markdown]: https://github.com/valich/intellij-markdown
[pegdown]: https://github.com/sirthias/pegdown
[spec.txt]: https://github.com/vsch/idea-multimarkdown/blob/master/test-data/performance/spec.md
[wrap.md]: https://github.com/vsch/idea-multimarkdown/blob/master/test-data/performance/wrap.md
| 0 |
aimacode/aima-java | Java implementation of algorithms from Russell And Norvig's Artificial Intelligence - A Modern Approach"" | null | # AIMA3e-Java (JDK 8+) [](https://travis-ci.org/aimacode/aima-java) [](https://mybinder.org/v2/gh/aimacode/aima-java/AIMA3e)
Java implementation of algorithms from [Russell](http://www.cs.berkeley.edu/~russell/) and [Norvig's](http://www.norvig.com/) [Artificial Intelligence - A Modern Approach 3rd Edition](http://aima.cs.berkeley.edu/). You can use this in conjunction with a course on AI, or for study on your own. We're looking for [solid contributors](https://github.com/aimacode/aima-java/wiki/AIMAJava-Contributing) to help.
### Getting Started Links
* [Overview of Project](https://github.com/aimacode/aima-java/wiki/AIMA3e-Overview)
* [Interested in Contributing](https://github.com/aimacode/aima-java/wiki/AIMAJava-Contributing)
* [Setting up your own workspace](https://github.com/aimacode/aima-java/wiki/AIMA3e-Workspace-Setup)
* [Comments on architecture and design](https://github.com/aimacode/aima-java/wiki/AIMA3e-Architecture-and-Design)
* [Demo Applications that can be run from your browser (unfortunately not up to date)](http://aimacode.github.io/aima-java/aima3e/aima3ejavademos.html)
* [Javadoc for the aima-core project (outdated)](http://aimacode.github.io/aima-java/aima3e/javadoc/aima-core/index.html)
* [Download the latest official (but outdated) version = 1.9.1 (Dec 18 2016)](https://github.com/aimacode/aima-java/releases/tag/aima3e-v1.9.1)
* Latest Maven Information (for integration as a third party library)<br>
```
<dependency>
<groupId>com.googlecode.aima-java</groupId>
<artifactId>aima-core</artifactId>
<version>3.0.0</version>
</dependency>
```
### Index of Implemented Algorithms
|Figure|Page|Name (in 3<sup>rd</sup> edition)|Code
| -------- |:--------:| :-----| :----- |
|2|34|Environment|[Environment](/aima-core/src/main/java/aima/core/agent/Environment.java)|
|2.1|35|Agent|[Agent](/aima-core/src/main/java/aima/core/agent/Agent.java)|
|2.3|36|Table-Driven-Vacuum-Agent|[TableDrivenVacuumAgent](/aima-core/src/main/java/aima/core/environment/vacuum/TableDrivenVacuumAgent.java)|
|2.7|47|Table-Driven-Agent|[TableDrivenAgentProgram](/aima-core/src/main/java/aima/core/agent/impl/aprog/TableDrivenAgentProgram.java)|
|2.8|48|Reflex-Vacuum-Agent|[ReflexVacuumAgent](/aima-core/src/main/java/aima/core/environment/vacuum/ReflexVacuumAgent.java)|
|2.10|49|Simple-Reflex-Agent|[SimpleReflexAgentProgram](/aima-core/src/main/java/aima/core/agent/impl/aprog/SimpleReflexAgentProgram.java)|
|2.12|51|Model-Based-Reflex-Agent|[ModelBasedReflexAgentProgram](/aima-core/src/main/java/aima/core/agent/impl/aprog/ModelBasedReflexAgentProgram.java)|
|3|66|Problem|[Problem](/aima-core/src/main/java/aima/core/search/framework/problem/Problem.java)|
|3.1|67|Simple-Problem-Solving-Agent|[SimpleProblemSolvingAgent](/aima-core/src/main/java/aima/core/search/agent/SimpleProblemSolvingAgent.java)|
|3.2|68|Romania|[SimplifiedRoadMapOfRomania](/aima-core/src/main/java/aima/core/environment/map/SimplifiedRoadMapOfRomania.java)|
|3.7|77|Tree-Search|[TreeSearch](/aima-core/src/main/java/aima/core/search/framework/qsearch/TreeSearch.java)|
|3.7|77|Graph-Search|[GraphSearch](/aima-core/src/main/java/aima/core/search/framework/qsearch/GraphSearch4e.java)|
|3.10|79|Node|[Node](/aima-core/src/main/java/aima/core/search/framework/Node.java)|
|3.11|82|Breadth-First-Search|[BreadthFirstSearch](/aima-core/src/main/java/aima/core/search/uninformed/BreadthFirstSearch.java)|
|3.14|84|Uniform-Cost-Search|[UniformCostSearch](/aima-core/src/main/java/aima/core/search/uninformed/UniformCostSearch.java)|
|3|85|Depth-first Search|[DepthFirstSearch](/aima-core/src/main/java/aima/core/search/uninformed/DepthFirstSearch.java)|
|3.17|88|Depth-Limited-Search|[DepthLimitedSearch](/aima-core/src/main/java/aima/core/search/uninformed/DepthLimitedSearch.java)|
|3.18|89|Iterative-Deepening-Search|[IterativeDeepeningSearch](/aima-core/src/main/java/aima/core/search/uninformed/IterativeDeepeningSearch.java)|
|3|90|Bidirectional search|[BidirectionalSearch](/aima-core/src/main/java/aima/core/search/framework/qsearch/BidirectionalSearch.java)|
|3|92|Best-First search|[BestFirstSearch](/aima-core/src/main/java/aima/core/search/informed/BestFirstSearch.java)|
|3|92|Greedy best-First search|[GreedyBestFirstSearch](/aima-core/src/main/java/aima/core/search/informed/GreedyBestFirstSearch.java)|
|3|93|A\* Search|[AStarSearch](/aima-core/src/main/java/aima/core/search/informed/AStarSearch.java)|
|3.26|99|Recursive-Best-First-Search |[RecursiveBestFirstSearch](/aima-core/src/main/java/aima/core/search/informed/RecursiveBestFirstSearch.java)|
|4.2|122|Hill-Climbing|[HillClimbingSearch](/aima-core/src/main/java/aima/core/search/local/HillClimbingSearch.java)|
|4.5|126|Simulated-Annealing|[SimulatedAnnealingSearch](/aima-core/src/main/java/aima/core/search/local/SimulatedAnnealingSearch.java)|
|4.8|129|Genetic-Algorithm|[GeneticAlgorithm](/aima-core/src/main/java/aima/core/search/local/GeneticAlgorithm.java)|
|4.11|136|And-Or-Graph-Search|[AndOrSearch](/aima-core/src/main/java/aima/core/search/nondeterministic/AndOrSearch.java)|
|4|147|Online search problem|[OnlineSearchProblem](/aima-core/src/main/java/aima/core/search/framework/problem/OnlineSearchProblem.java)|
|4.21|150|Online-DFS-Agent|[OnlineDFSAgent](/aima-core/src/main/java/aima/core/search/online/OnlineDFSAgent.java)|
|4.24|152|LRTA\*-Agent|[LRTAStarAgent](/aima-core/src/main/java/aima/core/search/online/LRTAStarAgent.java)|
|5.3|166|Minimax-Decision|[MinimaxSearch](/aima-core/src/main/java/aima/core/search/adversarial/MinimaxSearch.java)|
|5.7|170|Alpha-Beta-Search|[AlphaBetaSearch](/aima-core/src/main/java/aima/core/search/adversarial/AlphaBetaSearch.java)|
|6|202|CSP|[CSP](/aima-core/src/main/java/aima/core/search/csp/CSP.java)|
|6.1|204|Map CSP|[MapCSP](/aima-core/src/main/java/aima/core/search/csp/examples/MapCSP.java)|
|6.3|209|AC-3|[AC3Strategy](/aima-core/src/main/java/aima/core/search/csp/solver/inference/AC3Strategy.java)|
|6.5|215|Backtracking-Search|[AbstractBacktrackingSolver](/aima-core/src/main/java/aima/core/search/csp/solver/AbstractBacktrackingSolver.java)|
|6.8|221|Min-Conflicts|[MinConflictsSolver](/aima-core/src/main/java/aima/core/search/csp/solver/MinConflictsSolver.java)|
|6.11|224|Tree-CSP-Solver|[TreeCspSolver](/aima-core/src/main/java/aima/core/search/csp/solver/TreeCspSolver.java)|
|7|235|Knowledge Base|[KnowledgeBase](/aima-core/src/main/java/aima/core/logic/propositional/kb/KnowledgeBase.java)|
|7.1|236|KB-Agent|[KBAgent](/aima-core/src/main/java/aima/core/logic/propositional/agent/KBAgent.java)|
|7.7|244|Propositional-Logic-Sentence|[Sentence](/aima-core/src/main/java/aima/core/logic/propositional/parsing/ast/Sentence.java)|
|7.10|248|TT-Entails|[TTEntails](/aima-core/src/main/java/aima/core/logic/propositional/inference/TTEntails.java)|
|7|253|Convert-to-CNF|[ConvertToCNF](/aima-core/src/main/java/aima/core/logic/propositional/transformations/ConvertToCNF.java)|
|7.12|255|PL-Resolution|[PLResolution](/aima-core/src/main/java/aima/core/logic/propositional/inference/PLResolution.java)|
|7.15|258|PL-FC-Entails?|[PLFCEntails](/aima-core/src/main/java/aima/core/logic/propositional/inference/PLFCEntails.java)|
|7.17|261|DPLL-Satisfiable?|[DPLLSatisfiable](/aima-core/src/main/java/aima/core/logic/propositional/inference/DPLLSatisfiable.java)|
|7.18|263|WalkSAT|[WalkSAT](/aima-core/src/main/java/aima/core/logic/propositional/inference/WalkSAT.java)|
|7.20|270|Hybrid-Wumpus-Agent|[HybridWumpusAgent](/aima-core/src/main/java/aima/core/environment/wumpusworld/HybridWumpusAgent.java)|
|7.22|272|SATPlan|[SATPlan](/aima-core/src/main/java/aima/core/logic/propositional/inference/SATPlan.java)|
|9|323|Subst|[SubstVisitor](/aima-core/src/main/java/aima/core/logic/fol/SubstVisitor.java)|
|9.1|328|Unify|[Unifier](/aima-core/src/main/java/aima/core/logic/fol/Unifier.java)|
|9.3|332|FOL-FC-Ask|[FOLFCAsk](/aima-core/src/main/java/aima/core/logic/fol/inference/FOLFCAsk.java)|
|9.6|338|FOL-BC-Ask|[FOLBCAsk](/aima-core/src/main/java/aima/core/logic/fol/inference/FOLBCAsk.java)|
|9|345|CNF|[CNFConverter](/aima-core/src/main/java/aima/core/logic/fol/CNFConverter.java)|
|9|347|Resolution|[FOLTFMResolution](/aima-core/src/main/java/aima/core/logic/fol/inference/FOLTFMResolution.java)|
|9|354|Demodulation|[Demodulation](/aima-core/src/main/java/aima/core/logic/fol/inference/Demodulation.java)|
|9|354|Paramodulation|[Paramodulation](/aima-core/src/main/java/aima/core/logic/fol/inference/Paramodulation.java)|
|9|345|Subsumption|[SubsumptionElimination](/aima-core/src/main/java/aima/core/logic/fol/SubsumptionElimination.java)|
|10.9|383|Graphplan|[GraphPlan](/aima-core/src/main/java/aima/core/logic/planning/GraphPlanAlgorithm.java)|
|11.5|409|Hierarchical-Search|[HierarchicalSearchAlgorithm](/aima-core/src/main/java/aima/core/logic/planning/hierarchicalsearch/HierarchicalSearchAlgorithm.java)|
|11.8|414|Angelic-Search|---|
|13.1|484|DT-Agent|[DT-Agent](/aima-core/src/main/java/aima/core/agent/DTAgent.java)|
|13|484|Probability-Model|[ProbabilityModel](/aima-core/src/main/java/aima/core/probability/ProbabilityModel.java)|
|13|487|Probability-Distribution|[ProbabilityDistribution](/aima-core/src/main/java/aima/core/probability/ProbabilityDistribution.java)|
|13|490|Full-Joint-Distribution|[FullJointDistributionModel](/aima-core/src/main/java/aima/core/probability/full/FullJointDistributionModel.java)|
|14|510|Bayesian Network|[BayesianNetwork](/aima-core/src/main/java/aima/core/probability/bayes/BayesianNetwork.java)|
|14.9|525|Enumeration-Ask|[EnumerationAsk](/aima-core/src/main/java/aima/core/probability/bayes/exact/EnumerationAsk.java)|
|14.11|528|Elimination-Ask|[EliminationAsk](/aima-core/src/main/java/aima/core/probability/bayes/exact/EliminationAsk.java)|
|14.13|531|Prior-Sample|[PriorSample](/aima-core/src/main/java/aima/core/probability/bayes/approx/PriorSample.java)|
|14.14|533|Rejection-Sampling|[RejectionSampling](/aima-core/src/main/java/aima/core/probability/bayes/approx/RejectionSampling.java)|
|14.15|534|Likelihood-Weighting|[LikelihoodWeighting](/aima-core/src/main/java/aima/core/probability/bayes/approx/LikelihoodWeighting.java)|
|14.16|537|GIBBS-Ask|[GibbsAsk](/aima-core/src/main/java/aima/core/probability/bayes/approx/GibbsAsk.java)|
|15.4|576|Forward-Backward|[ForwardBackward](/aima-core/src/main/java/aima/core/probability/temporal/generic/ForwardBackward.java)|
|15|578|Hidden Markov Model|[HiddenMarkovModel](/aima-core/src/main/java/aima/core/probability/hmm/HiddenMarkovModel.java)|
|15.6|580|Fixed-Lag-Smoothing|[FixedLagSmoothing](/aima-core/src/main/java/aima/core/probability/hmm/exact/FixedLagSmoothing.java)|
|15|590|Dynamic Bayesian Network|[DynamicBayesianNetwork](/aima-core/src/main/java/aima/core/probability/bayes/DynamicBayesianNetwork.java)|
|15.17|598|Particle-Filtering|[ParticleFiltering](/aima-core/src/main/java/aima/core/probability/bayes/approx/ParticleFiltering.java)|
|16.9|632|Information-Gathering-Agent|[InformationGatheringAgent](/aima-core/src/main/java/aima/core/probability/InformationGatheringAgent.java)|
|17|647|Markov Decision Process|[MarkovDecisionProcess](/aima-core/src/main/java/aima/core/probability/mdp/MarkovDecisionProcess.java)|
|17.4|653|Value-Iteration|[ValueIteration](/aima-core/src/main/java/aima/core/probability/mdp/search/ValueIteration.java)|
|17.7|657|Policy-Iteration|[PolicyIteration](/aima-core/src/main/java/aima/core/probability/mdp/search/PolicyIteration.java)|
|17.9|663|POMDP-Value-Iteration|[POMDPValueIteration](/aima-core/src/main/java/aima/core/probability/mdp/search/POMDPValueIteration.java)|
|18.5|702|Decision-Tree-Learning|[DecisionTreeLearner](/aima-core/src/main/java/aima/core/learning/learners/DecisionTreeLearner.java)|
|18.8|710|Cross-Validation-Wrapper|[CrossValidation](/aima-core/src/main/java/aima/core/learning/inductive/CrossValidation.java)|
|18.11|717|Decision-List-Learning|[DecisionListLearner](/aima-core/src/main/java/aima/core/learning/learners/DecisionListLearner.java)|
|18.24|734|Back-Prop-Learning|[BackPropLearning](/aima-core/src/main/java/aima/core/learning/neural/BackPropLearning.java)|
|18.34|751|AdaBoost|[AdaBoostLearner](/aima-core/src/main/java/aima/core/learning/learners/AdaBoostLearner.java)|
|19.2|771|Current-Best-Learning|[CurrentBestLearning](/aima-core/src/main/java/aima/core/learning/knowledge/CurrentBestLearning.java)|
|19.3|773|Version-Space-Learning|[VersionSpaceLearning](/aima-core/src/main/java/aima/core/learning/knowledge/VersionSpaceLearning.java)|
|19.8|786|Minimal-Consistent-Det|[MinimalConsistentDet](/aima-core/src/main/java/aima/core/learning/knowledge/MinimalConsistentDet.java)|
|19.12|793|FOIL|[FOIL](https://github.com/aimacode/aima-java/blob/AIMA3e/aima-core/src/main/java/aima/core/learning/knowledge/FOIL.java)|
|21.2|834|Passive-ADP-Agent|[PassiveADPAgent](/aima-core/src/main/java/aima/core/learning/reinforcement/agent/PassiveADPAgent.java)|
|21.4|837|Passive-TD-Agent|[PassiveTDAgent](/aima-core/src/main/java/aima/core/learning/reinforcement/agent/PassiveTDAgent.java)|
|21.8|844|Q-Learning-Agent|[QLearningAgent](/aima-core/src/main/java/aima/core/learning/reinforcement/agent/QLearningAgent.java)|
|22.1|871|HITS|[HITS](/aima-core/src/main/java/aima/core/nlp/ranking/HITS.java)|
|23.5|894|CYK-Parse|[CYK](/aima-core/src/main/java/aima/core/nlp/parsing/CYK.java)|
|25.9|982|Monte-Carlo-Localization|[MonteCarloLocalization](/aima-core/src/main/java/aima/core/robotics/MonteCarloLocalization.java)|
### Index of implemented notebooks
|Chapter No|Name |Status (in 3<sup>rd</sup> edition)|Status (in 4<sup>th</sup> edition)
| -------- |:--------:| :-----| :----- |
|3| [Solving Problems by Searching](https://github.com/aimacode/aima-java/blob/AIMA3e/notebooks/ClassicalSearch.ipynb)| In Progress| Not started|
|6| [Constraint Satisfaction Problems](https://github.com/aimacode/aima-java/blob/AIMA3e/notebooks/ProbabilisticReasoning.ipynb) |In Progress|---|
|12| [Knowledge Representation](https://github.com/aimacode/aima-java/blob/AIMA3e/notebooks/KnowledgeRepresentation.ipynb)|Done|---|
|13| [Quantifying Uncertainty](https://github.com/aimacode/aima-java/blob/AIMA3e/notebooks/QuantifyingUncertainty.ipynb) |Done | --- |
|14| [Probabilistic Reasoning](https://github.com/aimacode/aima-java/blob/AIMA3e/notebooks/ProbabilisticReasoning.ipynb)|In Progress| ---|
Before starting to work on a new notebook:
1. Open a new issue with the following heading: **Notebook: Chapter Name - Version **. Check that the issue is not assigned to anyone.
2. Mention a topics list of what you will be implementing in the notebook for that particular chapter. You can iteratively refine the list once you start working.
3. Start a discussion on what can go in that particular notebook.
### "---" indicates algorithms yet to be implemented.
### Index of data structures
Here is a table of the data structures yet to be implemented.
|Fig|Page|Name (in book)|Code|
| -------- |:--------:| :-----| :----- |
|9.8|341|Append|---|
|10.1|369|AIR-CARGO-TRANSPORT-PROBLEM|---|
|10.2|370|SPARE-TIRE-PROBLEM|---|
|10.3|371|BLOCKS-WORLD |---|
|10.7|380|HAVE-CAKE-AND-EAT-CAKE-TOO-PROBLEM|---|
|11.1|402|JOB-SHOP-SCHEDULING-PROBLEM|---|
|11.4|407|REFINEMENT-HIGH-LEVEL-ACTIONS|---|
|23.6|895|SENTENCE-TREE|---|
|29.1|1062|POWERS-OF-2|---|
| 0 |
google/google-java-format | Reformats Java source code to comply with Google Java Style. | formatter java | # google-java-format
`google-java-format` is a program that reformats Java source code to comply with
[Google Java Style][].
[Google Java Style]: https://google.github.io/styleguide/javaguide.html
## Using the formatter
### From the command-line
[Download the formatter](https://github.com/google/google-java-format/releases)
and run it with:
```
java -jar /path/to/google-java-format-${GJF_VERSION?}-all-deps.jar <options> [files...]
```
The formatter can act on whole files, on limited lines (`--lines`), on specific
offsets (`--offset`), passing through to standard-out (default) or altered
in-place (`--replace`).
To reformat changed lines in a specific patch, use
[`google-java-format-diff.py`](https://github.com/google/google-java-format/blob/master/scripts/google-java-format-diff.py).
***Note:*** *There is no configurability as to the formatter's algorithm for
formatting. This is a deliberate design decision to unify our code formatting on
a single format.*
### IntelliJ, Android Studio, and other JetBrains IDEs
A
[google-java-format IntelliJ plugin](https://plugins.jetbrains.com/plugin/8527)
is available from the plugin repository. To install it, go to your IDE's
settings and select the `Plugins` category. Click the `Marketplace` tab, search
for the `google-java-format` plugin, and click the `Install` button.
The plugin will be disabled by default. To enable it in the current project, go
to `File→Settings...→google-java-format Settings` (or `IntelliJ
IDEA→Preferences...→Other Settings→google-java-format Settings` on macOS) and
check the `Enable google-java-format` checkbox. (A notification will be
presented when you first open a project offering to do this for you.)
To enable it by default in new projects, use `File→Other Settings→Default
Settings...`.
When enabled, it will replace the normal `Reformat Code` and `Optimize Imports`
actions.
#### IntelliJ JRE Config
The google-java-format plugin uses some internal classes that aren't available
without extra configuration. To use the plugin, go to `Help→Edit Custom VM
Options...` and paste in these lines:
```
--add-exports=jdk.compiler/com.sun.tools.javac.api=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.code=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.file=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.parser=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.tree=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.util=ALL-UNNAMED
```
Once you've done that, restart the IDE.
### Eclipse
The latest version of the `google-java-format` Eclipse plugin can be downloaded
from the [releases page](https://github.com/google/google-java-format/releases).
Drop it into the Eclipse
[drop-ins folder](http://help.eclipse.org/neon/index.jsp?topic=%2Forg.eclipse.platform.doc.isv%2Freference%2Fmisc%2Fp2_dropins_format.html)
to activate the plugin.
The plugin adds a `google-java-format` formatter implementation that can be
configured in `Window > Preferences > Java > Code Style > Formatter > Formatter
Implementation`.
### Third-party integrations
* Visual Studio Code
* [google-java-format-for-vs-code](https://marketplace.visualstudio.com/items?itemName=JoseVSeb.google-java-format-for-vs-code)
* Gradle plugins
* [spotless](https://github.com/diffplug/spotless/tree/main/plugin-gradle#google-java-format)
* [sherter/google-java-format-gradle-plugin](https://github.com/sherter/google-java-format-gradle-plugin)
* Apache Maven plugins
* [spotless](https://github.com/diffplug/spotless/tree/main/plugin-maven#google-java-format)
* [spotify/fmt-maven-plugin](https://github.com/spotify/fmt-maven-plugin)
* [talios/googleformatter-maven-plugin](https://github.com/talios/googleformatter-maven-plugin)
* [Cosium/maven-git-code-format](https://github.com/Cosium/maven-git-code-format):
A maven plugin that automatically deploys google-java-format as a
pre-commit git hook.
* SBT plugins
* [sbt/sbt-java-formatter](https://github.com/sbt/sbt-java-formatter)
* [Github Actions](https://github.com/features/actions)
* [googlejavaformat-action](https://github.com/axel-op/googlejavaformat-action):
Automatically format your Java files when you push on github
### as a library
The formatter can be used in software which generates java to output more
legible java code. Just include the library in your maven/gradle/etc.
configuration.
`google-java-format` uses internal javac APIs for parsing Java source. The
following JVM flags are required when running on JDK 16 and newer, due to
[JEP 396: Strongly Encapsulate JDK Internals by Default](https://openjdk.java.net/jeps/396):
```
--add-exports=jdk.compiler/com.sun.tools.javac.api=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.code=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.file=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.parser=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.tree=ALL-UNNAMED
--add-exports=jdk.compiler/com.sun.tools.javac.util=ALL-UNNAMED
```
#### Maven
```xml
<dependency>
<groupId>com.google.googlejavaformat</groupId>
<artifactId>google-java-format</artifactId>
<version>${google-java-format.version}</version>
</dependency>
```
#### Gradle
```groovy
dependencies {
implementation 'com.google.googlejavaformat:google-java-format:$googleJavaFormatVersion'
}
```
You can then use the formatter through the `formatSource` methods. E.g.
```java
String formattedSource = new Formatter().formatSource(sourceString);
```
or
```java
CharSource source = ...
CharSink output = ...
new Formatter().formatSource(source, output);
```
Your starting point should be the instance methods of
`com.google.googlejavaformat.java.Formatter`.
## Building from source
```
mvn install
```
## Contributing
Please see [the contributors guide](CONTRIBUTING.md) for details.
## License
```text
Copyright 2015 Google Inc.
Licensed under the Apache License, Version 2.0 (the "License"); you may not
use this file except in compliance with the License. You may obtain a copy of
the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
License for the specific language governing permissions and limitations under
the License.
```
| 0 |
a466350665/smart-sso | springboot SSO 单点登录,OAuth2实现,支持App登录,支持分布式 | java oauth2 springboot sso | # smart-sso
[](http://opensource.org/licenses/MIT)
[](https://github.com/a466350665/smart-sso/pulls)
[](https://github.com/a466350665/smart-sso)
[](https://github.com/a466350665/smart-sso)
QQ交流群:454343484🈵、769134727
## 简述
smart-sso使用当下最流行的SpringBoot技术,基于OAuth2认证授权协议,为您构建一个易理解、高可用、高扩展性的分布式单点登录应用基层。
## 相关文档
- [smart-sso单点登录(一):简介](https://blog.csdn.net/a466350665/article/details/54140411)
- [smart-sso单点登录(二):部署文档](http://blog.csdn.net/a466350665/article/details/79628553)
- [smart-sso单点登录(三):App登录支持](https://blog.csdn.net/a466350665/article/details/109742638)
- [smart-sso单点登录(四):引入redis支持分布式](https://blog.csdn.net/a466350665/article/details/109388429)
## 组织结构
```lua
smart-sso
├── smart-sso-client -- 客户端依赖包
├── smart-sso-client-redis -- 客户端依赖包,分布式redis支持
├── smart-sso-demo -- 客户端
├── smart-sso-server -- 服务端
```
## 单点登录原理
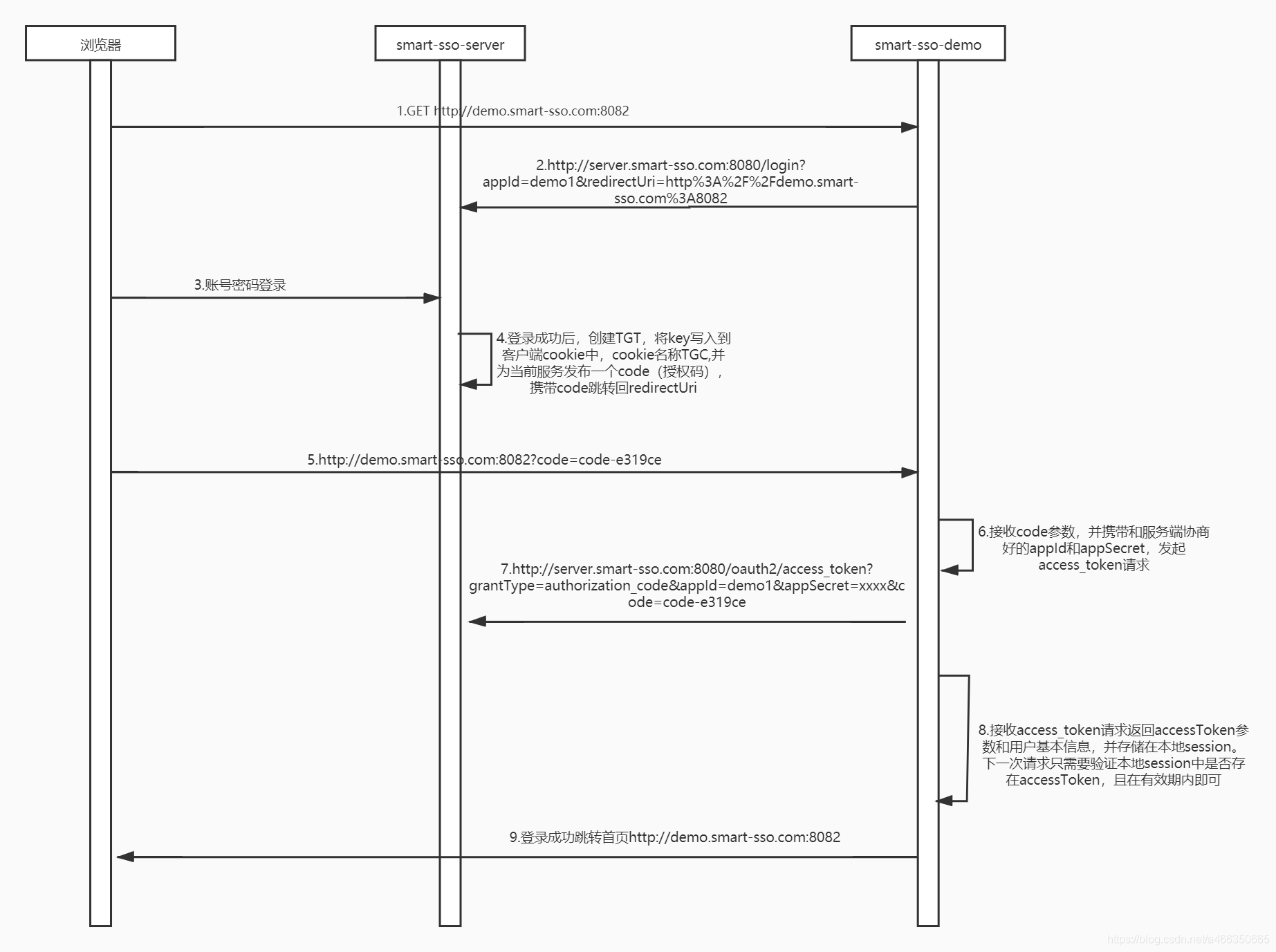
## 单点退出原理
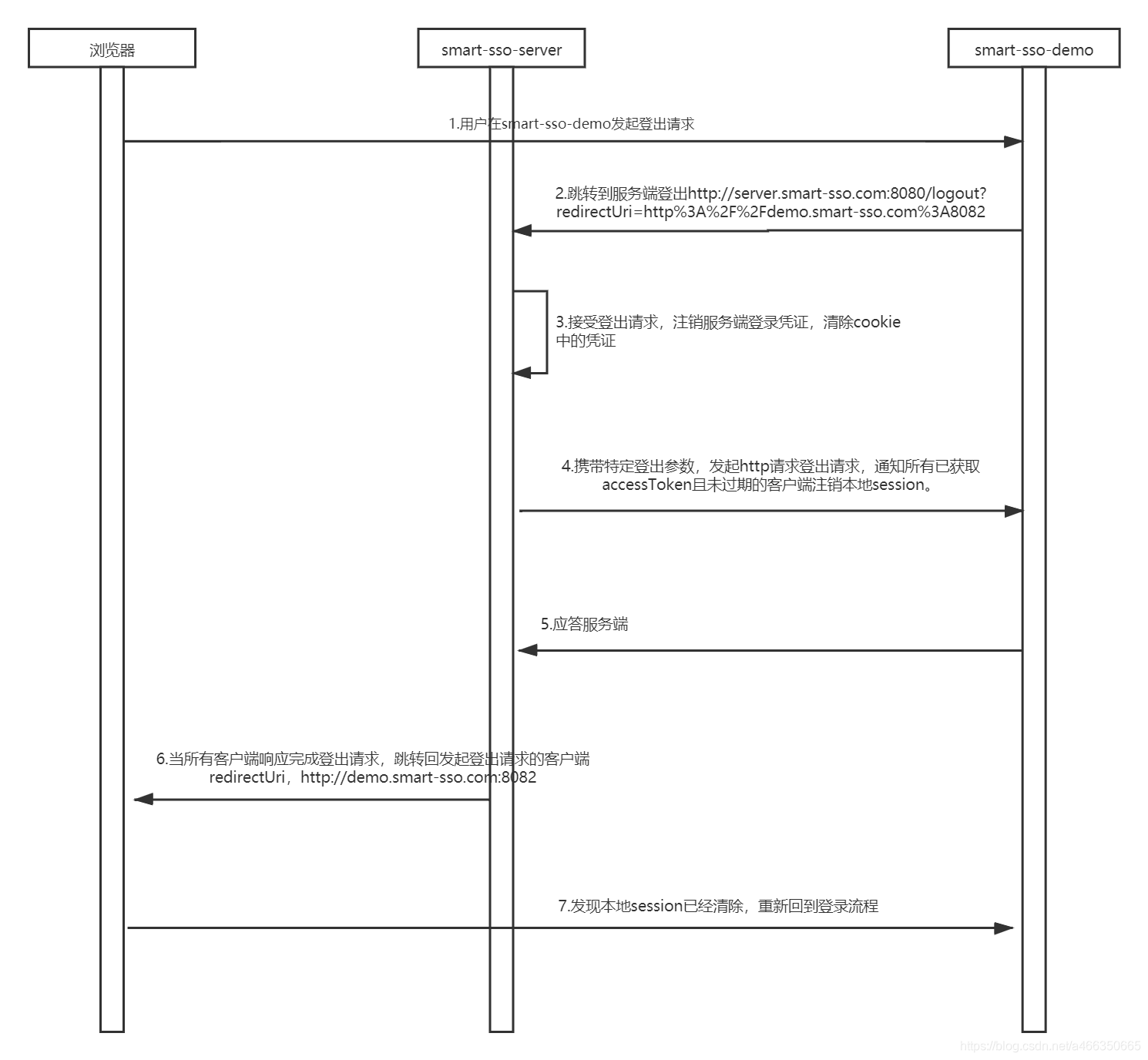
## 效果展示
### 单点登录页
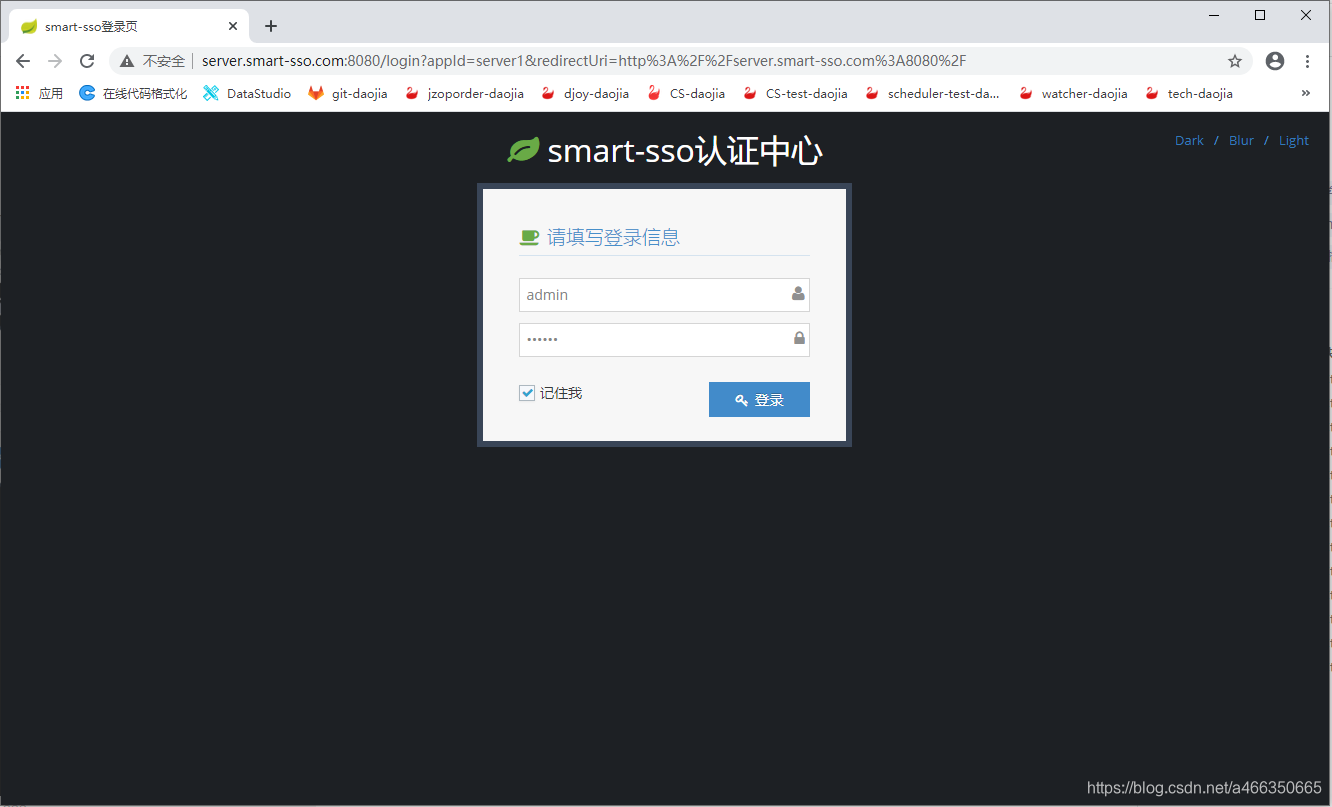
### 服务端登录成功页
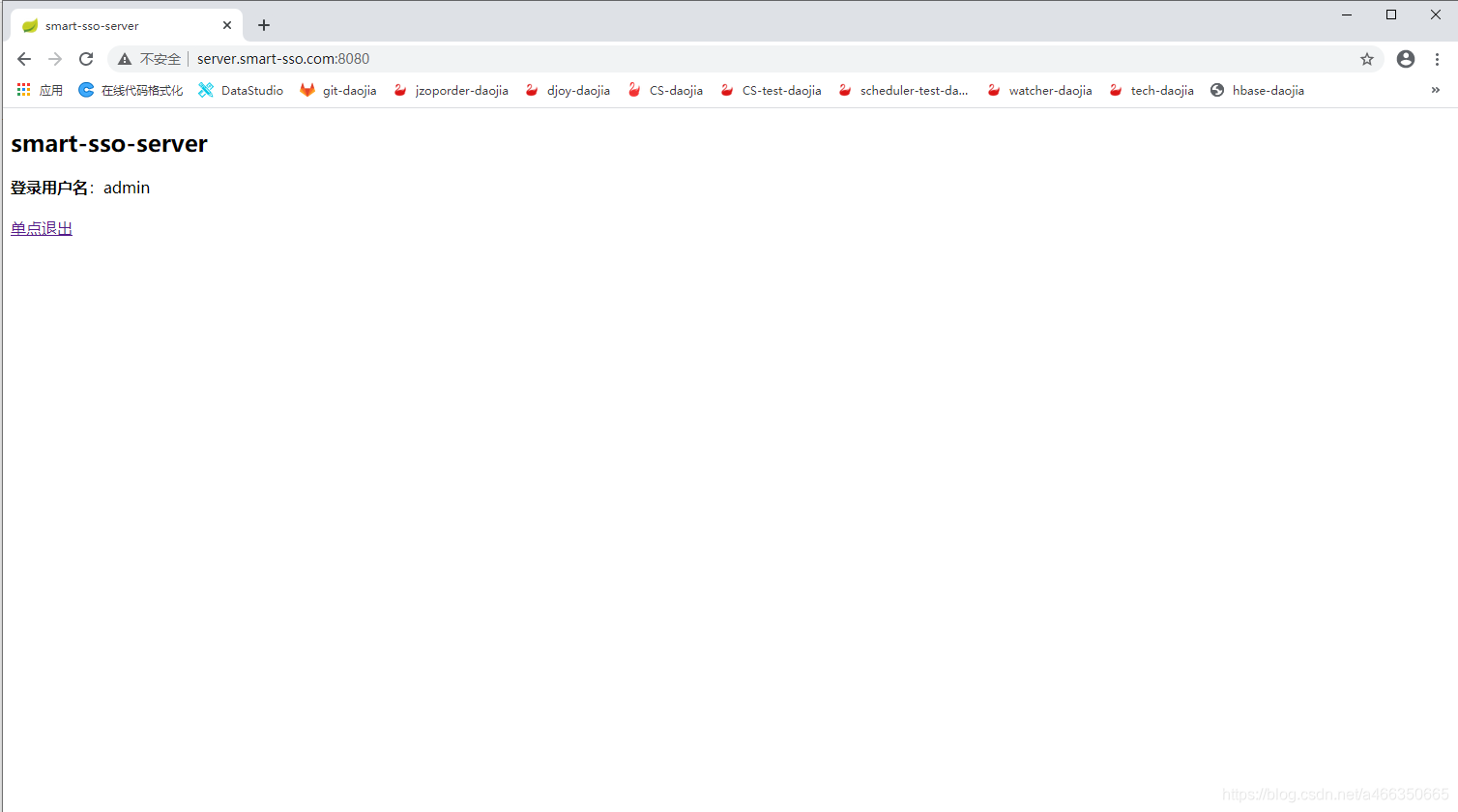
### 客户端登录成功页
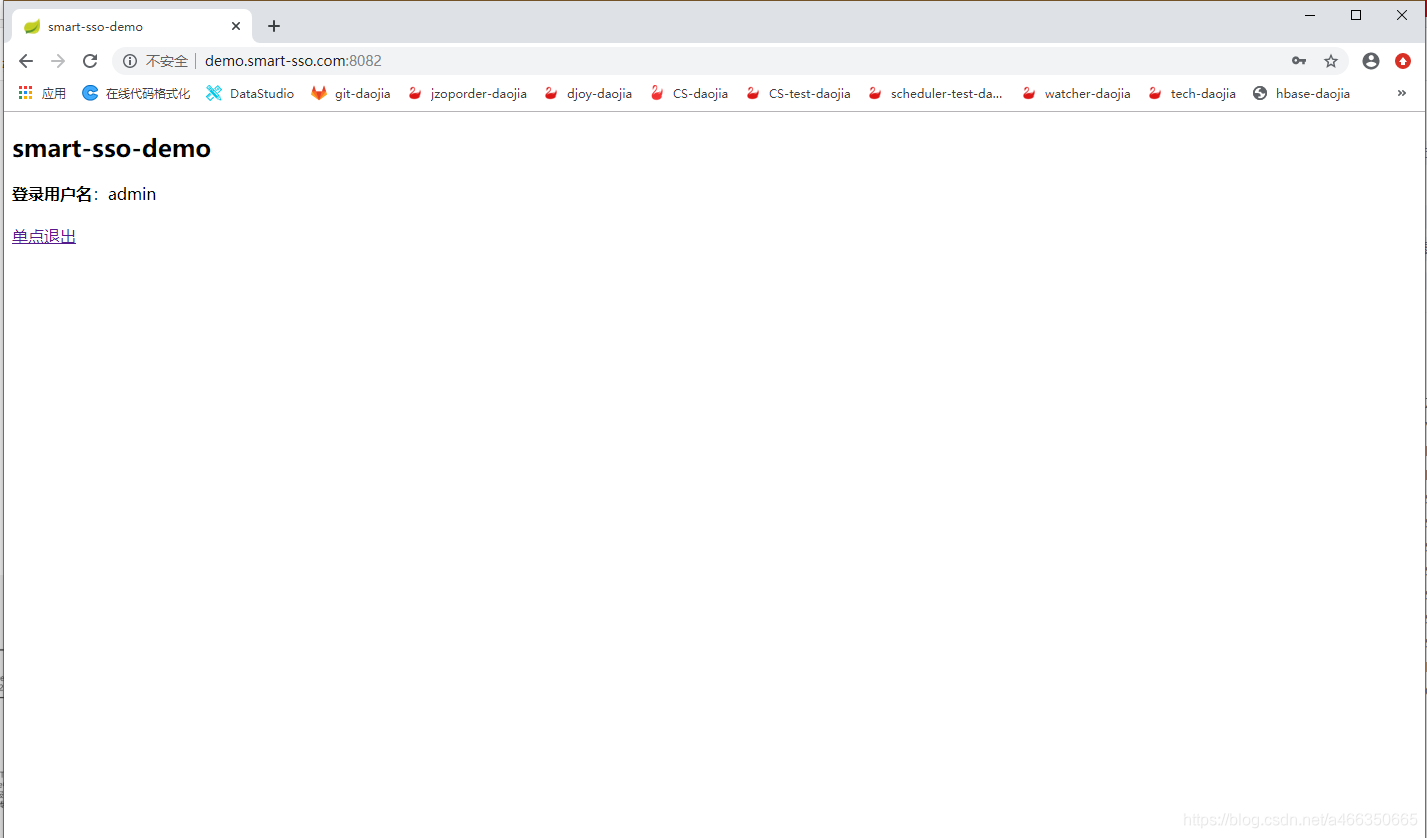 | 0 |
wxiaoqi/Spring-Cloud-Platform | 🔥🔥🔥国内首个Spring Cloud微服务化RBAC的管理平台,核心采用Spring Boot 2.4、Spring Cloud 2020.0.0 & Alibaba,前端采用d2-admin中台框架。 🔝 🔝 记得上边点个star 关注更新 | jwt spring-boot spring-cloud spring-cloud-alibaba spring-cloud-gateway spring-cloud-loadbalancer | ### Cloud-Platform
Cloud-Platform是国内首个基于`Spring
Cloud`微`服务`化`开发平台`,具有统一授权、认证后台管理系统,其中包含具备用户管理、资源权限管理、网关API
管理等多个模块,支持多业务系统并行开发,可以作为后端服务的开发脚手架。代码简洁,架构清晰,适合学习和直接项目中使用。
核心技术采用`Spring Boot
2.4.1`、`Spring Cloud (2020.0.0)`以及`Spring Cloud Alibaba 2.2.4`
相关核心组件,采用`Nacos`注册和配置中心,集成流量卫兵`Sentinel`,前端采用`vue-element-admin`组件,`Elastic Search`自行集成。
### QQ群号:169824183(1)、592462556(2)、661605461(3)
### 公众号

#### 开源用户登记,宣传用:[点击打开](https://gitee.com/geek_qi/cloud-platform/issues/II9SP)
#### 最新更新日志,[点击查看](https://gitee.com/geek_qi/cloud-platform/wikis/Cloud-Platform更新日志?sort_id=320050)
#### 基础文档,[点击查看](https://gitee.com/geek_qi/cloud-platform/blob/master/dev-doc.md)
# 模块说明

### 架构摘要
#### 中台化前端
集成`d2admin`中台化前端,优化前端架构和功能布局,支撑中台服务化的应用开发。
#### JWT鉴权
通过`JWT`的方式来进行用户认证和信息传递,保证服务之间用户无状态的传递。
#### 监控
利用Spring Boot Admin 来监控各个独立Service的运行状态;利用Hystrix Dashboard来实时查看接口的运行状态和调用频率等。
#### 负载均衡
将服务保留的rest进行代理和网关控制,除了平常经常使用的node.js、nginx外,Spring Cloud系列的zuul和ribbon,可以帮我们进行正常的网关管控和负载均衡。其中扩展和借鉴国外项目的扩展基于JWT的`Zuul限流插件`,方面进行限流。
#### 服务注册与调用
基于`Nacos`来实现的服务注册与调用,在Spring Cloud中使用Feign, 我们可以做到使用HTTP请求远程服务时能与调用本地方法一样的编码体验,开发者完全感知不到这是远程方法,更感知不到这是个HTTP请求。
#### 熔断与流控
集成阿里`Sentinel`进行接口流量控制,通过熔断和降级处理避免服务之间的调用“雪崩”。
------
## 功能截图
### 基本功能
# 功能截图













## License
Apache License Version 2.0
| 0 |
lets-blade/blade | :rocket: Lightning fast and elegant mvc framework for Java8 | blade java8 mvc-framework netty4 restful template-engine | <p align="center">
<a href="https://lets-blade.com"><img src="https://i.loli.net/2018/09/18/5ba0cd93c710e.png" width="650"/></a>
</p>
<p align="center">Based on <code>Java8</code> + <code>Netty4</code> to create a lightweight, high-performance, simple and elegant Web framework 😋</p>
<p align="center">Spend <b>1 hour</b> to learn it to do something interesting, a tool in addition to the other available frameworks.</p>
<p align="center">
🐾 <a href="#quick-start" target="_blank">Quick Start</a> |
🌚 <a href="https://lets-blade.github.io/" target="_blank">Documentation</a> |
:green_book: <a href="https://www.baeldung.com/blade" target="_blank">Guidebook</a> |
💰 <a href="https://lets-blade.github.io/donate" target="_blank">Donate</a> |
🇨🇳 <a href="README_CN.md">简体中文</a>
</p>
<p align="center">
<a href="https://travis-ci.org/lets-blade/blade"><img src="https://img.shields.io/travis/lets-blade/blade.svg?style=flat-square"></a>
<a href="http://search.maven.org/#search%7Cga%7C1%7Cblade-core"><img src="https://img.shields.io/maven-central/v/com.hellokaton/blade-core.svg?style=flat-square"></a>
<a href="LICENSE"><img src="https://img.shields.io/badge/license-Apache%202-4EB1BA.svg?style=flat-square"></a>
<a class="badge-align" href="https://www.codacy.com/gh/lets-blade/blade/dashboard"><img src="https://app.codacy.com/project/badge/Grade/1eff0e30bf694402ac1b0ebe587bfa5a"/></a>
<a href="https://gitter.im/lets-blade/blade"><img src="https://badges.gitter.im/hellokaton/blade.svg?style=flat-square"></a>
<a href="https://www.codetriage.com/lets-blade/blade"><img src="https://www.codetriage.com/lets-blade/blade/badges/users.svg"></a>
</p>
***
## What Is Blade?
`Blade` is a pursuit of simple, efficient Web framework, so that `JavaWeb` development becomes even more powerful, both in performance and flexibility.
If you like to try something interesting, I believe you will love it.
If you think it's good, you can support it with a [star](https://github.com/lets-blade/blade/stargazers) or by [donating](https://ko-fi.com/hellokaton) :blush:
## Features
* [x] A new generation MVC framework that doesn't depend on other libraries
* [x] Get rid of SSH's bloated, modular design
* [x] Source is less than `500kb`, learning it is also simple
* [x] RESTful-style routing design
* [x] Template engine support, view development more flexible
* [x] High performance, 100 concurrent qps 20w/s
* [x] Run the `JAR` package to open the web service
* [x] Streams-style API
* [x] `CSRF` and `XSS` defense
* [x] `Basic Auth` and `Authorization`
* [x] Supports plug-in extensions
* [x] Support webjars resources
* [x] Tasks based on `cron` expressions
* [x] Built-in a variety of commonly used middleware
* [x] Built-in Response output
* [x] JDK8 +
## Overview
» Simplicity: The design is simple, easy to understand and doesn't introduce many layers between you and the standard library. The goal of this project is that the users should be able to understand the whole framework in a single day.<br/>
» Elegance: `blade` supports the RESTful style routing interface, has no invasive interceptors and provides the writing of a DSL grammar.<br/>
» Easy deploy: supports `maven` package `jar` file running.<br/>
## Quick Start
Create a basic `Maven` or `Gradle` project.
> Do not create a `webapp` project, Blade does not require much trouble.
Run with `Maven`:
```xml
<dependency>
<groupId>com.hellokaton</groupId>
<artifactId>blade-core</artifactId>
<version>2.1.2.RELEASE</version>
</dependency>
```
or `Gradle`:
```sh
compile 'com.hellokaton:blade-core:2.1.2.RELEASE'
```
Write the `main` method and the `Hello World`:
```java
public static void main(String[] args) {
Blade.create().get("/", ctx -> ctx.text("Hello Blade")).start();
}
```
Open http://localhost:9000 in your browser to see your first `Blade` application!
## Contents
- [**`Register Route`**](#register-route)
- [**`HardCode`**](#hardCode)
- [**`Controller`**](#controller)
- [**`Request Parameter`**](#request-parameter)
- [**`URL Parameter`**](#URL-parameter)
- [**`Form Parameter`**](#form-parameter)
- [**`Path Parameter`**](#path-parameter)
- [**`Body Parameter`**](#body-parameter)
- [**`Parse To Model`**](#parse-to-model)
- [**`Get Environment`**](#get-environment)
- [**`Get Header`**](#get-header)
- [**`Get Cookie`**](#get-cookie)
- [**`Static Resource`**](#static-resource)
- [**`Upload File`**](#upload-file)
- [**`Download File`**](#download-file)
- [**`Set Session`**](#set-session)
- [**`Render To Browser`**](#render-to-browser)
- [**`Render Response`**](#render-json)
- [**`Render Text`**](#render-text)
- [**`Render Html`**](#render-html)
- [**`Render Template`**](#render-template)
- [**`Default Template`**](#default-template)
- [**`Jetbrick Template`**](#jetbrick-template)
- [**`Redirects`**](#redirects)
- [**`Write Cookie`**](#write-cookie)
- [**`Web Hook`**](#web-hook)
- [**`Logging`**](#logging)
- [**`Basic Auth`**](#basic-auth)
- [**`Change Server Port`**](#change-server-port)
- [**`Configuration SSL`**](#configuration-ssl)
- [**`Custom Exception Handler`**](#custom-exception-handler)
## Register Route
### HardCode
```java
public static void main(String[] args) {
// Create multiple routes GET, POST, PUT, DELETE using Blade instance
Blade.create()
.get("/user/21", getting)
.post("/save", posting)
.delete("/remove", deleting)
.put("/putValue", putting)
.start();
}
```
### `Controller`
```java
@Path
public class IndexController {
@GET("/login")
public String login(){
return "login.html";
}
@POST(value = "/login", responseType = ResponseType.JSON)
public RestResponse doLogin(RouteContext ctx){
// do something
return RestResponse.ok();
}
}
```
## Request Parameter
### URL Parameter
**Using RouteContext**
```java
public static void main(String[] args) {
Blade.create().get("/user", ctx -> {
Integer age = ctx.queryInt("age");
System.out.println("age is:" + age);
}).start();
}
```
**Using `@Query` annotation**
```java
@GET("/user")
public void savePerson(@Query Integer age){
System.out.println("age is:" + age);
}
```
Test it with sample data from the terminal
```bash
curl -X GET http://127.0.0.1:9000/user?age=25
```
### Form Parameter
Here is an example:
**Using RouteContext**
```java
public static void main(String[] args) {
Blade.create().get("/user", ctx -> {
Integer age = ctx.fromInt("age");
System.out.println("age is:" + age);
}).start();
}
```
**Using `@Form` Annotation**
```java
@POST("/save")
public void savePerson(@Form String username, @Form Integer age){
System.out.println("username is:" + username + ", age is:" + age);
}
```
Test it with sample data from the terminal
```bash
curl -X POST http://127.0.0.1:9000/save -F username=jack -F age=16
```
### Path Parameter
**Using RouteContext**
```java
public static void main(String[] args) {
Blade blade = Blade.create();
// Create a route: /user/:uid
blade.get("/user/:uid", ctx -> {
Integer uid = ctx.pathInt("uid");
ctx.text("uid : " + uid);
});
// Create two parameters route
blade.get("/users/:uid/post/:pid", ctx -> {
Integer uid = ctx.pathInt("uid");
Integer pid = ctx.pathInt("pid");
String msg = "uid = " + uid + ", pid = " + pid;
ctx.text(msg);
});
// Start blade
blade.start();
}
```
**Using `@PathParam` Annotation**
```java
@GET("/users/:username/:page")
public void userTopics(@PathParam String username, @PathParam Integer page){
System.out.println("username is:" + usernam + ", page is:" + page);
}
```
Test it with sample data from the terminal
```bash
curl -X GET http://127.0.0.1:9000/users/hellokaton/2
```
### Body Parameter
```java
public static void main(String[] args) {
Blade.create().post("/body", ctx -> {
System.out.println("body string is:" + ctx.bodyToString());
}).start();
}
```
**Using `@Body` Annotation**
```java
@POST("/body")
public void readBody(@Body String data){
System.out.println("data is:" + data);
}
```
Test it with sample data from the terminal
```bash
curl -X POST http://127.0.0.1:9000/body -d '{"username":"hellokaton","age":22}'
```
### Parse To Model
This is the `User` model.
```java
public class User {
private String username;
private Integer age;
// getter and setter
}
```
**By Annotation**
```java
@POST("/users")
public void saveUser(@Form User user) {
System.out.println("user => " + user);
}
```
Test it with sample data from the terminal
```bash
curl -X POST http://127.0.0.1:9000/users -F username=jack -F age=16
```
**Custom model identification**
```java
@POST("/users")
public void saveUser(@Form(name="u") User user) {
System.out.println("user => " + user);
}
```
Test it with sample data from the terminal
```bash
curl -X POST http://127.0.0.1:9000/users -F u[username]=jack -F u[age]=16
```
**Body Parameter To Model**
```java
@POST("/body")
public void body(@Body User user) {
System.out.println("user => " + user);
}
```
Test it with sample data from the terminal
```bash
curl -X POST http://127.0.0.1:9000/body -d '{"username":"hellokaton","age":22}'
```
## Get Environment
```java
Environment environment = WebContext.blade().environment();
String version = environment.get("app.version", "0.0.1");
```
## Get Header
**By Context**
```java
@GET("header")
public void readHeader(RouteContext ctx){
System.out.println("Host => " + ctx.header("Host"));
// get useragent
System.out.println("UserAgent => " + ctx.userAgent());
// get client ip
System.out.println("Client Address => " + ctx.address());
}
```
**By Annotation**
```java
@GET("header")
public void readHeader(@Header String host){
System.out.println("Host => " + host);
}
```
## Get Cookie
**By Context**
```java
@GET("cookie")
public void readCookie(RouteContext ctx){
System.out.println("UID => " + ctx.cookie("UID"));
}
```
**By Annotation**
```java
@GET("cookie")
public void readCookie(@Cookie String uid){
System.out.println("Cookie UID => " + uid);
}
```
## Static Resource
Blade builds a few static resource catalog, as long as you will save the resource file in the static directory under the classpath, and then browse http://127.0.0.1:9000/static/style.css
If you want to customize the static resource URL
```java
Blade.create().addStatics("/mydir");
```
Of course you can also specify it in the configuration file. `application.properties` (location in classpath)
```bash
mvc.statics=/mydir
```
## Upload File
**By Request**
```java
@POST("upload")
public void upload(Request request){
request.fileItem("img").ifPresent(fileItem -> {
fileItem.moveTo(new File(fileItem.getFileName()));
});
}
```
**By Annotation**
```java
@POST("upload")
public void upload(@Multipart FileItem fileItem){
// Save to new path
fileItem.moveTo(new File(fileItem.getFileName()));
}
```
## Download File
```java
@GET(value = "/download", responseType = ResponseType.STREAM)
public void download(Response response) throws IOException {
response.write("abcd.pdf", new File("146373013842336153820220427172437.pdf"));
}
```
**If you want to preview certain files in your browser**
```java
@GET(value = "/preview", responseType = ResponseType.PREVIEW)
public void preview(Response response) throws IOException {
response.write(new File("146373013842336153820220427172437.pdf"));
}
```
## Set Session
The session is disabled by default, you must enable the session.
```java
Blade.create()
.http(HttpOptions::enableSession)
.start(Application.class, args);
```
> 💡 It can also be enabled using a configuration file,`http.session.enabled=true`
```java
public void login(Session session){
// if login success
session.attribute("login_key", SOME_MODEL);
}
```
## Render To Browser
### Render Response
**By Context**
```java
@GET("users/json")
public void printJSON(RouteContext ctx){
User user = new User("hellokaton", 18);
ctx.json(user);
}
```
**By Annotation**
This form looks more concise 😶
```java
@GET(value = "/users/json", responseType = ResponseType.JSON)
public User printJSON(){
return new User("hellokaton", 18);
}
```
### Render Text
```java
@GET("text")
public void printText(RouteContext ctx){
ctx.text("I Love Blade!");
}
```
or
```java
@GET(value = "/text", responseType = ResponseType.TEXT)
public String printText(RouteContext ctx){
return "I Love Blade!";
}
```
### Render Html
```java
@GET("html")
public void printHtml(RouteContext ctx){
ctx.html("<center><h1>I Love Blade!</h1></center>");
}
```
or
```java
@GET(value = "/html", responseType = ResponseType.HTML)
public String printHtml(RouteContext ctx){
return "<center><h1>I Love Blade!</h1></center>";
}
```
## Render Template
By default all template files are in the templates directory; in most of the cases you do not need to change it.
### Default Template
By default, Blade uses the built-in template engine, which is very simple. In a real-world web project, you can try several other extensions.
```java
public static void main(String[] args) {
Blade.create().get("/hello", ctx -> {
ctx.attribute("name", "hellokaton");
ctx.render("hello.html");
}).start(Hello.class, args);
}
```
The `hello.html` template
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello Page</title>
</head>
<body>
<h1>Hello, ${name}</h1>
</body>
</html>
```
### Jetbrick Template
**Config Jetbrick Template**
Create a `BladeLoader` class and load some config
```java
@Bean
public class TemplateConfig implements BladeLoader {
@Override
public void load(Blade blade) {
blade.templateEngine(new JetbrickTemplateEngine());
}
}
```
Write some data for the template engine to render
```java
public static void main(String[] args) {
Blade.create().get("/hello", ctx -> {
User user = new User("hellokaton", 50);
ctx.attribute("user", user);
ctx.render("hello.html");
}).start(Hello.class, args);
}
```
The `hello.html` template
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello Page</title>
</head>
<body>
<h1>Hello, ${user.username}</h1>
#if(user.age > 18)
<p>Good Boy!</p>
#else
<p>Gooood Baby!</p>
#end
</body>
</html>
```
[Render API](http://static.javadoc.io/com.hellokaton/blade-core/2.1.2.RELEASE/com/hellokaton/blade/mvc/http/Response.html#render-com.ModelAndView-)
## Redirects
```java
@GET("redirect")
public void redirectToGithub(RouteContext ctx){
ctx.redirect("https://github.com/hellokaton");
}
```
[Redirect API](http://static.javadoc.io/com.hellokaton/blade-core/2.1.2.RELEASE/com/hellokaton/blade/mvc/http/Response.html#redirect-java.lang.String-)
## Write Cookie
```java
@GET("write-cookie")
public void writeCookie(RouteContext ctx){
ctx.cookie("hello", "world");
ctx.cookie("UID", "22", 3600);
}
```
[Cookie API](http://static.javadoc.io/com.hellokaton/blade-core/2.1.2.RELEASE/com/hellokaton/blade/mvc/http/Response.html#cookie-java.lang.String-java.lang.String-)
## Web Hook
`WebHook` is the interface in the Blade framework that can be intercepted before and after the execution of the route.
```java
public static void main(String[] args) {
// All requests are exported before execution before
Blade.create().before("/*", ctx -> {
System.out.println("before...");
}).start();
}
```
## Logging
Blade uses slf4j-api as logging interface, the default implementation of a simple log package (modified from simple-logger); if you need complex logging you can also use a custom library, you only need to exclude the `blade-log` from the dependencies.
```java
private static final Logger log = LoggerFactory.getLogger(Hello.class);
public static void main(String[] args) {
log.info("Hello Info, {}", "2017");
log.warn("Hello Warn");
log.debug("Hello Debug");
log.error("Hello Error");
}
```
## Basic Auth
Blade includes a few middleware, like Basic Authentication; of course, it can also be customized to achieve more complex goals.
```java
public static void main(String[] args) {
Blade.create().use(new BasicAuthMiddleware()).start();
}
```
Specify the user name and password in the `application.properties` configuration file.
```bash
http.auth.username=admin
http.auth.password=123456
```
## Change Server Port
There are three ways to modify the port: hard coding it, in a configuration file, and through a command line parameter.
**Hard Coding**
```java
Blade.create().listen(9001).start();
```
**Configuration For `application.properties`**
```bash
server.port=9001
```
**Command Line**
```bash
java -jar blade-app.jar --server.port=9001
```
## Configuration SSL
**Configuration For `application.properties`**
```bash
server.ssl.enable=true
server.ssl.cert-path=cert.pem
server.ssl.private-key-path=private_key.pem
server.ssl.private-key-pass=123456
```
** Configuration using INettySslCustomizer **
```bash
#Specify any properties your customizer needs, for example
server.ssl.enable=true
server.keystore.path=fully qualified path
server.keystore.type=PKCS12
server.keystore.password=mypass
server.keystore.alias=optional alias
```
* Create your implementation of INettySslCustomizer
* Register it with Blade class
```java
MyNettySslCustomizer nc = new MyNettySslCustomizer();
Blade.create()
.setNettySslCustomizer(nc)
.start(App.class, args);
}
```
Sample implementation of INettySslCustomizer
```java
public class MyNettySSLCustomizer implements INettySslCustomizer {
public SslContext getCustomSslContext(Blade blade) {
SslContext sslctx = null;
// get my custom properties from the environment
String keystoreType = blade.getEnv("server.keystore.type", null);
String keystorePath = blade.getEnv("server.keystore.path", null);
String keystorePass = blade.getEnv("server.keystore.password", null);
if (verifyKeystore(keystoreType, keystorePath, keystorePass)) {
try (FileInputStream instream = new FileInputStream(new File(keystorePath))) {
// verify I can load store and password is valid
KeyStore keystore = KeyStore.getInstance(keystoreType);
char[] storepw = keystorePass.toCharArray();
keystore.load(instream, storepw);
KeyManagerFactory kmf = KeyManagerFactory.getInstance(KeyManagerFactory.getDefaultAlgorithm());
kmf.init(keystore, storepw);
sslctx = SslContextBuilder.forServer(kmf).build();
} catch (Exception ex) {
log.error("Keystore validation failed " + ex.getMessage());
}
} else {
log.error("Unable to load keystore, sslContext creation failed.");
}
return sslctx;
}
```
## Custom Exception Handler
Blade has an exception handler already implemented by default; if you need to deal with custom exceptions, you can do it like follows.
```java
@Bean
public class GlobalExceptionHandler extends DefaultExceptionHandler {
@Override
public void handle(Exception e) {
if (e instanceof CustomException) {
CustomException customException = (CustomException) e;
String code = customException.getCode();
// do something
} else {
super.handle(e);
}
}
}
```
Besides looking easy, the features above are only the tip of the iceberg, and there are more surprises to see in the documentation and sample projects:
+ [Blade Demos](https://github.com/lets-blade/blade-demos)
+ [Awesome Blade](https://github.com/lets-blade/awesome-blade)
## Change Logs
[See Here](https://lets-blade.com/about/change-logs)
## Contact
- Twitter: [hellokaton](https://twitter.com/hellokaton)
- Mail: hellokaton@gmail.com
## Contributors
Thanks goes to these wonderful people

Contributions of any kind are welcome!
## Licenses
Please see [Apache License](LICENSE)
| 0 |
aws/serverless-java-container | A Java wrapper to run Spring, Spring Boot, Jersey, and other apps inside AWS Lambda. | api api-gateway api-server aws aws-lambda jersey rest-api serverless sparkjava sparkjava-framework spring | # Serverless Java container [](https://github.com/aws/serverless-java-container/actions) [](https://maven-badges.herokuapp.com/maven-central/com.amazonaws.serverless/aws-serverless-java-container) [](https://gitter.im/aws/serverless-java-container)
The `aws-serverless-java-container` makes it easy to run Java applications written with frameworks such as [Spring](https://spring.io/), [Spring Boot](https://projects.spring.io/spring-boot/), [Apache Struts](http://struts.apache.org/), [Jersey](https://jersey.java.net/), or [Spark](http://sparkjava.com/) in [AWS Lambda](https://aws.amazon.com/lambda/).
Serverless Java Container natively supports API Gateway's proxy integration models for requests and responses, you can create and inject custom models for methods that use custom mappings.
Currently the following versions are maintained:
| Version | Branch | Java Enterprise support | Spring versions | JAX-RS/ Jersey version | Struts support | Spark support |
|---------|--------|-------------------------|-----------------|------------------------|----------------|---------------|
| 1.x | [1.x](https://github.com/aws/serverless-java-container/tree/1.x) | Java EE (javax.*) | 5.x (Boot 2.x) | 2.x | :white_check_mark: | :white_check_mark: |
| 2.x | [main](https://github.com/aws/serverless-java-container/tree/main) | Jakarta EE (jakarta.*) | 6.x (Boot 3.x) | 3.x | :x: | :x: |
Follow the quick start guides in [our wiki](https://github.com/aws/serverless-java-container/wiki) to integrate Serverless Java Container with your project:
* [Spring quick start](https://github.com/aws/serverless-java-container/wiki/Quick-start---Spring)
* [Spring Boot 2 quick start](https://github.com/aws/serverless-java-container/wiki/Quick-start---Spring-Boot2)
* [Spring Boot 3 quick start](https://github.com/aws/serverless-java-container/wiki/Quick-start---Spring-Boot3)
* [Apache Struts quick start](https://github.com/aws/serverless-java-container/wiki/Quick-start---Struts)
* [Jersey quick start](https://github.com/aws/serverless-java-container/wiki/Quick-start---Jersey)
* [Spark quick start](https://github.com/aws/serverless-java-container/wiki/Quick-start---Spark)
Below is the most basic AWS Lambda handler example that launches a Spring application. You can also take a look at the [samples](https://github.com/aws/serverless-java-container/tree/master/samples) in this repository, our main wiki page includes a [step-by-step guide](https://github.com/aws/serverless-java-container/wiki#deploying-the-sample-applications) on how to deploy the various sample applications using Maven and [SAM](https://github.com/awslabs/serverless-application-model).
```java
public class StreamLambdaHandler implements RequestStreamHandler {
private static final SpringLambdaContainerHandler<AwsProxyRequest, AwsProxyResponse> handler;
static {
try {
handler = SpringLambdaContainerHandler.getAwsProxyHandler(PetStoreSpringAppConfig.class);
} catch (ContainerInitializationException e) {
// if we fail here. We re-throw the exception to force another cold start
e.printStackTrace();
throw new RuntimeException("Could not initialize Spring framework", e);
}
}
@Override
public void handleRequest(InputStream inputStream, OutputStream outputStream, Context context)
throws IOException {
handler.proxyStream(inputStream, outputStream, context);
}
}
```
## Public Examples
### Blogs
- [Re-platforming Java applications using the updated AWS Serverless Java Container](https://aws.amazon.com/blogs/compute/re-platforming-java-applications-using-the-updated-aws-serverless-java-container/)
### Workshops
- [Java on AWS Lambda](https://catalog.workshops.aws/java-on-aws-lambda) From Serverful to Serverless Java with AWS Lambda in 2 hours
### Videos
- [Spring on AWS Lambda](https://www.youtube.com/watch?v=A1rYiHTy9Lg&list=PLCOG9xkUD90IDm9tcY-5nMK6X6g8SD-Sz) YouTube Playlist from [@plantpowerjames](https://twitter.com/plantpowerjames)
### Java samples with different frameworks
- [Dagger, Micronaut, Quarkus, Spring Boot](https://github.com/aws-samples/serverless-java-frameworks-samples/)
| 0 |
synthetichealth/synthea | Synthetic Patient Population Simulator | fhir health-data simulation synthea synthetic-data synthetic-population | # Synthea<sup>TM</sup> Patient Generator  [](https://codecov.io/gh/synthetichealth/synthea)
Synthea<sup>TM</sup> is a Synthetic Patient Population Simulator. The goal is to output synthetic, realistic (but not real), patient data and associated health records in a variety of formats.
Read our [wiki](https://github.com/synthetichealth/synthea/wiki) and [Frequently Asked Questions](https://github.com/synthetichealth/synthea/wiki/Frequently-Asked-Questions) for more information.
Currently, Synthea<sup>TM</sup> features include:
- Birth to Death Lifecycle
- Configuration-based statistics and demographics (defaults with Massachusetts Census data)
- Modular Rule System
- Drop in [Generic Modules](https://github.com/synthetichealth/synthea/wiki/Generic-Module-Framework)
- Custom Java rules modules for additional capabilities
- Primary Care Encounters, Emergency Room Encounters, and Symptom-Driven Encounters
- Conditions, Allergies, Medications, Vaccinations, Observations/Vitals, Labs, Procedures, CarePlans
- Formats
- HL7 FHIR (R4, STU3 v3.0.1, and DSTU2 v1.0.2)
- Bulk FHIR in ndjson format (set `exporter.fhir.bulk_data = true` to activate)
- C-CDA (set `exporter.ccda.export = true` to activate)
- CSV (set `exporter.csv.export = true` to activate)
- CPCDS (set `exporter.cpcds.export = true` to activate)
- Rendering Rules and Disease Modules with Graphviz
## Developer Quick Start
These instructions are intended for those wishing to examine the Synthea source code, extend it or build the code locally. Those just wishing to run Synthea should follow the [Basic Setup and Running](https://github.com/synthetichealth/synthea/wiki/Basic-Setup-and-Running) instructions instead.
### Installation
**System Requirements:**
Synthea<sup>TM</sup> requires Java JDK 11 or newer. We strongly recommend using a Long-Term Support (LTS) release of Java, 11 or 17, as issues may occur with more recent non-LTS versions.
To clone the Synthea<sup>TM</sup> repo, then build and run the test suite:
```
git clone https://github.com/synthetichealth/synthea.git
cd synthea
./gradlew build check test
```
### Changing the default properties
The default properties file values can be found at `src/main/resources/synthea.properties`.
By default, synthea does not generate CCDA, CPCDA, CSV, or Bulk FHIR (ndjson). You'll need to
adjust this file to activate these features. See the [wiki](https://github.com/synthetichealth/synthea/wiki)
for more details, or use our [guided customizer tool](https://synthetichealth.github.io/spt/#/customizer).
### Generate Synthetic Patients
Generating the population one at a time...
```
./run_synthea
```
Command-line arguments may be provided to specify a state, city, population size, or seed for randomization.
```
run_synthea [-s seed] [-p populationSize] [state [city]]
```
Full usage info can be printed by passing the `-h` option.
```
$ ./run_synthea -h
> Task :run
Usage: run_synthea [options] [state [city]]
Options: [-s seed]
[-cs clinicianSeed]
[-p populationSize]
[-r referenceDate as YYYYMMDD]
[-g gender]
[-a minAge-maxAge]
[-o overflowPopulation]
[-c localConfigFilePath]
[-d localModulesDirPath]
[-i initialPopulationSnapshotPath]
[-u updatedPopulationSnapshotPath]
[-t updateTimePeriodInDays]
[-f fixedRecordPath]
[-k keepMatchingPatientsPath]
[--config*=value]
* any setting from src/main/resources/synthea.properties
Examples:
run_synthea Massachusetts
run_synthea Alaska Juneau
run_synthea -s 12345
run_synthea -p 1000
run_synthea -s 987 Washington Seattle
run_synthea -s 21 -p 100 Utah "Salt Lake City"
run_synthea -g M -a 60-65
run_synthea -p 10 --exporter.fhir.export=true
run_synthea --exporter.baseDirectory="./output_tx/" Texas
```
Some settings can be changed in `./src/main/resources/synthea.properties`.
Synthea<sup>TM</sup> will output patient records in C-CDA and FHIR formats in `./output`.
### Synthea<sup>TM</sup> GraphViz
Generate graphical visualizations of Synthea<sup>TM</sup> rules and modules.
```
./gradlew graphviz
```
### Concepts and Attributes
Generate a list of concepts (used in the records) or attributes (variables on each patient).
```
./gradlew concepts
./gradlew attributes
```
# License
Copyright 2017-2023 The MITRE Corporation
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
| 0 |
freerouting/freerouting | Advanced PCB auto-router | auto-route auto-router autoroute autorouter autorouting dsn help-wanted kicad pcb pcb-design router routing routing-engine specctra | <p align="center">
<img src="https://raw.githubusercontent.com/freerouting/freerouting/master/design/social_preview/freerouting_social_preview_1280x960_v2.png" alt="Freerouting" title="Freerouting" align="center">
</p>
<h1 align="center">Freerouting</h1>
<h5 align="center">Freerouting is an advanced autorouter for all PCB programs that support the standard Specctra or Electra DSN interface.</h5>
<p align="center">
<a href="https://github.com/freerouting/freerouting/releases"><img src="https://img.shields.io/github/v/release/freerouting/freerouting" alt="Release version" /></a>
<img src="https://img.shields.io/github/downloads/freerouting/freerouting/v1.9.0/total" alt="Downloads"/>
<img src="https://img.shields.io/github/downloads/freerouting/freerouting/total" alt="Downloads"/>
<a href="LICENSE"><img src="https://img.shields.io/github/license/freerouting/freerouting" alt="License"/></a>
</p>
<h3 align="center">:point_right: This project needs JAVA and UI/UX volunteers! Contact @andrasfuchs for details! :point_left:</h3>
<br/>
<br/>
[Installers for Windows and Linux can be downloaded here.](https://github.com/freerouting/freerouting/releases)
## Introduction
This software can be used together with all host PCB design software systems containing a standard Specctra or Electra DSN interface. It imports .DSN files generated by the Specctra interface of the host system and exports .SES Specctra session files.
Although the software can be used for manual routing in 90 degree, 45 degree and free angle modes, it's main focus is on autorouting.
### Getting started
You can run Freerouting as a standalone application.
1) After launching freerouting.jar, a window appears prompting you to select your exported .DSN design file.
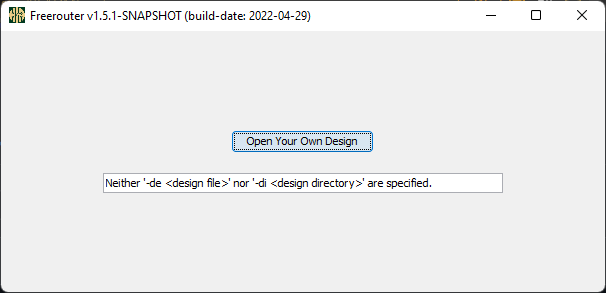
2) After opening a design you can start the autorouter with the button in the toolbar on top of the board window.
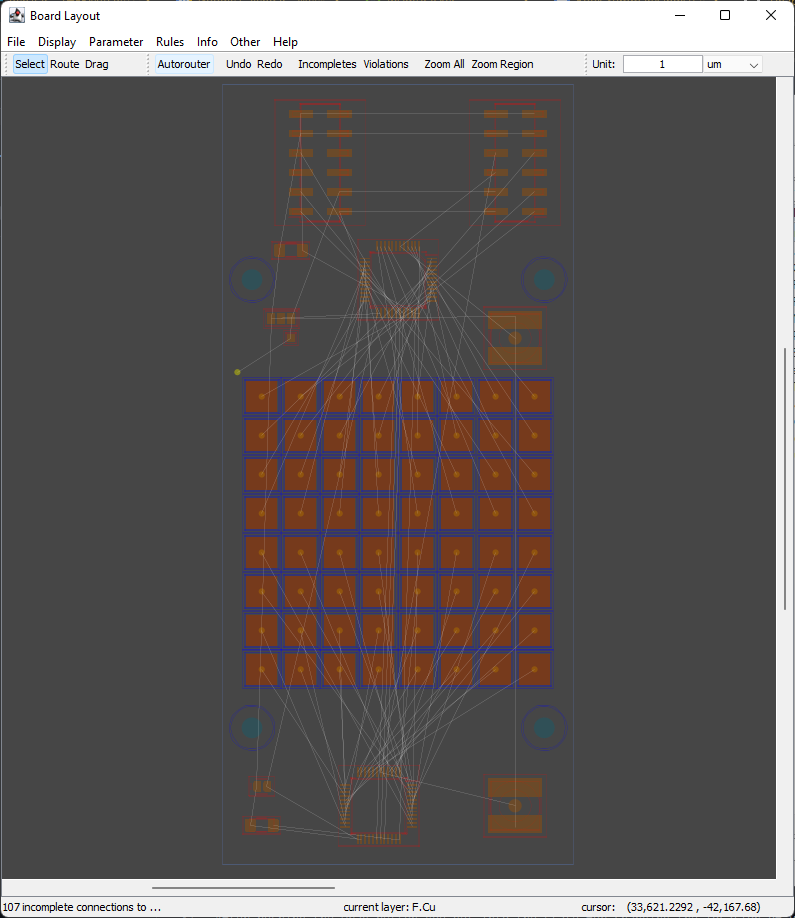
3) While autorouter is running you can follow the progress both visually in the board editor and numerically in the footer.
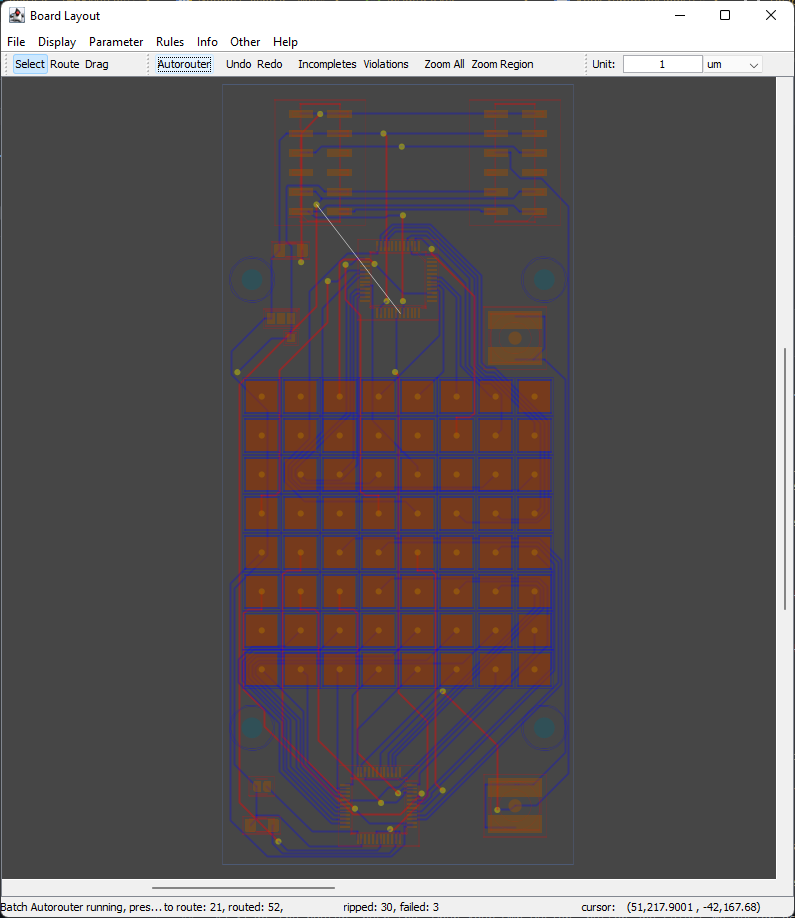
4) You are going to have a short summary when it is finished.
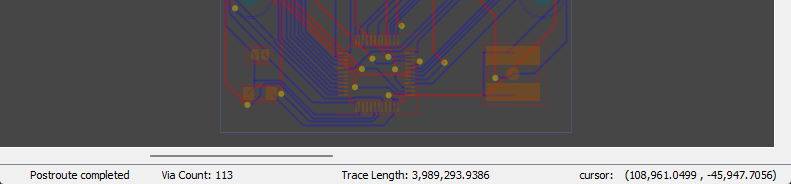
5) You can now save your routed board as a .SES file in the File / Export Specctra Session File menu.
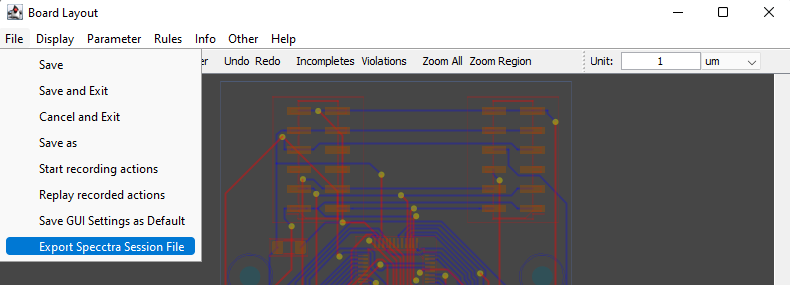
If you use [KiCad](#additional-steps-for-users-of-kicad), [Autodesk EAGLE](#additional-steps-for-users-of-autodesk-eagle), [Target3001!](#additional-steps-for-users-of-target-3001) or [pcb-rnd](#additional-steps-for-users-of-pcb-rnd), [click here for their integrations](/docs/integrations.md).
## Using the command line arguments
Freerouter was designed as a GUI program, but it also can function as a command line tool. Typically you would have an input file (e.g. Specctra DSN) that you exported from you EDA (e.g. KiCad). If this file has unconnected routes, you would want to wire those with autorouter, and save the result in a format that you can then import back into your EDA.
The following command line arguments are supported by freerouter:
* -de [design input file]: loads up a Specctra .dsn file at startup.
* -di [design input directory]: if the GUI is used, this sets the default folder for the open design dialogs.
* -dr [design rules file]: reads the rules from a previously saved .rules file.
* -do [design output file]: saves a Specctra board (.dsn), a Specctra session file (.ses) or Eagle session script file (.scr) when the routing is finished.
* -mp [number of passes]: sets the upper limit of the number of auto-router passes that will be performed.
* -l [language]: "en" for English, "de" for German, "zh" for Simplified Chinese, otherwise it's the system default. English is set by default for unsupported languages.
* -host [host_name host_version]: sets the name of the host process, if it was run as an external library or plugin.
* -mt [number of threads]: sets thread pool size for route optimization. The default is one less than the number of logical processors in the system. Set it to 0 to disable route optimization.
* -oit [percentage]: stops the route optimizer if the improvement drops below a certain percentage threshold per pass. Default is 0.1%, and `-oit 0` means to continue improving until it is interrupted by the user or it runs out of options to test.
* -us [greedy | global | hybrid]: sets board updating strategy for route optimization: greedy, global optimal or hybrid. The default is greedy. When hybrid is selected, another option "hr" specifies hybrid ratio.
* -hr [m:n]: sets hybrid ratio in the format of #_global_optiomal_passes:#_prioritized_passes. The default is 1:1. It's only effective when hybrid strategy is selected.
* -is [sequential | random | prioritized]: sets item selection strategy for route optimization: sequential, random, prioritized. The default is prioritized. Prioritied strategy selects items based on scores calculated in previous round.
* -inc [net class names, separated by commas]: auto-router ignores the listed net classes, eg. `-inc GND,VCC` will not try to wire components that are either in the "GND" or in the "VCC" net class.
* -im: saves intermediate steps in version-specific binary format. This allows to user to resume the interrupted optimization from the last checkpoint. Turned off by default.
* -dct [seconds]: dialog confirmation timeout. Sets the timeout of the dialogs that start a default action in x seconds. 20 seconds by default.
* -da: disable anonymous analytics.
* -dl: disable logging.
* -help: shows help.
A complete command line looks something like this if your are using PowerShell on Windows:
```powershell
java.exe -jar freerouting-1.9.0.jar -de MyBoard.dsn -do MyBoard.ses -mp 100 -dr MyBoard.rules
```
This would read the _MyBoard.dsn_ file, do the auto-routing with the parameters defined in _MyBoard.rules_ for the maximum of 100 passes, and then save the result into the _MyBoard.ses_ file.
## Running Freerouting using Java JRE
There are only installers for Windows x64 and Linux x64. Fortunatelly though the platform independent .JAR files can be run on the other systems, if the matching Java runtime is installed.
You will need the following steps to make it work:
1. Get the current JAR release from our [Releases page](https://github.com/freerouting/freerouting/releases)
2. Install [Java JRE](https://adoptium.net/temurin/releases/)
* Select your operating system and architecture
* Select `JRE` as package type
* Select `21` as version
4. Run the downloaded JAR file using the installed java
```powershell
java -jar freerouting-1.9.0.jar
```
(macOS: please note that you can't start Freerouting from the Mac Finder, you must use the Mac Terminal instead!)
## Contributing
We ❤️ all our contributors; this project wouldn’t be what it is without you!
If you want to help out, please consider replying to [issues](https://github.com/freerouting/freerouting/issues), creating new ones, or even send your fixes and improvements as [pull requests](https://github.com/freerouting/freerouting/pulls). [Our developer documentation](/docs/developer.md) can help you with the technicalities.
| 0 |
PureWriter/desktop | Pure Writer Desktop | null | # Pure Writer Desktop
https://writer.drakeet.com/desktop
### [PureWriter](https://play.google.com/store/apps/details?id=com.drakeet.purewriter) for Android:
<a href='https://play.google.com/store/apps/details?id=com.drakeet.purewriter&utm_source=global_co&utm_medium=prtnr&utm_content=Mar2515&utm_campaign=PartBadge&pcampaignid=MKT-Other-global-all-co-prtnr-py-PartBadge-Mar2515-1'><img alt='Get it on Google Play' src='https://play.google.com/intl/en_us/badges/images/generic/en_badge_web_generic.png' width=200 height=77/></a>
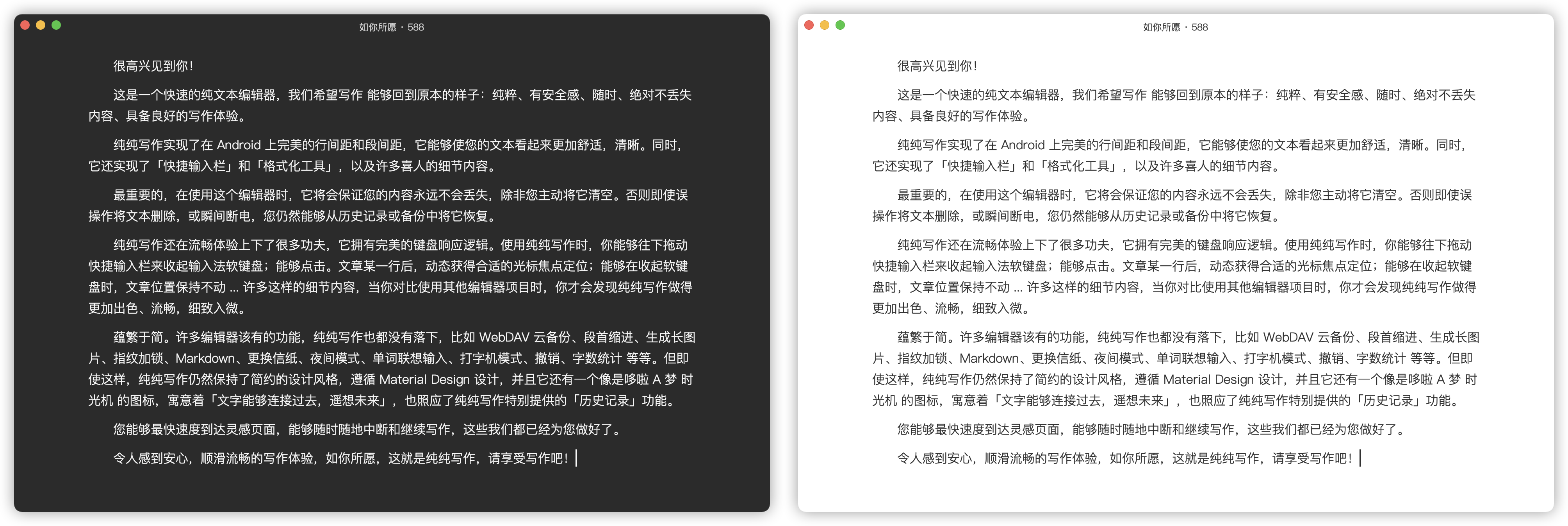
### Build
```
./gradlew jfxNative
```
# Download
https://github.com/purewriter/desktop/releases
### LICENSE
=======
Copyright (C) 2019 Drakeet <drakeet@drakeet.com>
This file is part of Pure Writer Desktop
Pure Writer Desktop is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
rebase-server is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with rebase-server. If not, see <http://www.gnu.org/licenses/>.
| 0 |
git-commit-id/git-commit-id-maven-plugin | Maven plugin which includes build-time git repository information into an POJO / *.properties). Make your apps tell you which version exactly they were built from! Priceless in large distributed deployments... :-) | build-automation continuous-delivery git maven maven-plugin versioning | maven git commit id plugin
==================================
[](https://github.com/git-commit-id/git-commit-id-maven-plugin/actions)
[](https://coveralls.io/github/git-commit-id/git-commit-id-maven-plugin?branch=master)
[](https://search.maven.org/artifact/io.github.git-commit-id/git-commit-id-maven-plugin)
git-commit-id-maven-plugin is a plugin quite similar to [Build Number Maven Plugin](https://www.mojohaus.org/buildnumber-maven-plugin/index.html) for example but as the Build Number plugin at the time when I started this plugin only supported CVS and SVN, something had to be done.
I had to quickly develop a Git version of such a plugin. For those who don't know the plugin, it basically helps you with the following tasks and answers related questions
* Which version had the bug? Is that deployed already?
* Make your distributed deployment aware of versions
* Validate if properties are set as expected
If you are more interested in the different use-cases, feel free to [read about them in more detail](docs/use-cases.md).
Quicklinks (all relevant documentation)
==================
* [Use case documentation](docs/use-cases.md)
* [Using the plugin documentation (all details for configuration, properties, ...)](docs/using-the-plugin.md)
* [A more technical documentation on how to use the leverage the generated properties from this plugin](docs/using-the-plugin-in-more-depth.md)
* [A general documentation for git describe (usefull feature in this plugin, if you are not familiar with the command)](docs/git-describe.md)
* [All configuration options are documented inside `GitCommitIdMojo.java` as Javadoc](src/main/java/pl/project13/maven/git/GitCommitIdMojo.java#L98)
* [Frequently Asked Question (FAQ)](docs/faq.md)
* [Contributing](CONTRIBUTING.md)
Getting the plugin
==================
The plugin **is available from Maven Central** ([see here](https://search.maven.org/artifact/io.github.git-commit-id/git-commit-id-maven-plugin)), so you don't have to configure any additional repositories to use this plugin.
A detailed description of using the plugin is available in the [Using the plugin](docs/using-the-plugin.md) document. All you need to do in the basic setup is to include that plugin definition in your `pom.xml`.
For more advanced users we also prepared a [guide to provide a brief overview of the more advanced configurations](docs/using-the-plugin.md)... read on!
Relocation of the Project
------------------------
Newer version (5.x.x or more recent) are available via
```xml
<groupId>io.github.git-commit-id</groupId>
<artifactId>git-commit-id-maven-plugin</artifactId>
```
older version (4.x.x or older) are available via:
```xml
<groupId>pl.project13.maven</groupId>
<artifactId>git-commit-id-plugin</artifactId>
```
Versions
--------
The current version is **8.0.2** ([changelist](https://github.com/git-commit-id/git-commit-id-maven-plugin/issues?q=milestone%3A8.0.2)).
You can check the available versions by visiting [search.maven.org](https://search.maven.org/artifact/io.github.git-commit-id/git-commit-id-maven-plugin), though using the newest is obviously the best choice.
Plugin compatibility with Java
-------------------------------
Here is an overview of the current plugin compatibility with Java
| Plugin Version | Required Java Version |
| --------------- | ---------------------:|
| 2.1.X | Java 1.6 |
| 2.2.X | Java 1.7 |
| 3.X.X | Java 1.8 |
| 4.X.X | Java 1.8 |
| 5.X.X | Java 11 |
| 6.X.X | Java 11 |
| 7.X.X | Java 11 |
| 8.X.X | Java 11 |
Plugin compatibility with Maven
-----------------------------
Even though this plugin tries to be compatible with every Maven version there are some known limitations with specific versions. Here is a list that tries to outline the current state of the art:
| Plugin Version | Minimal Required Maven version |
|----------------|:----------------------------------------------------------:|
| 2.1.X | Maven 2.2.1 up to v2.1.13; Maven 3.1.1 for any later 2.1.X |
| 2.2.X | Maven 3.1.1 up to v2.2.3; Maven 3.0 for any later 2.2.X |
| 3.X.X | Maven 3.0 |
| 4.X.X | Maven 3.0 |
| 5.X.X | Maven 3.1.0-alpha-1 |
| 6.X.X | Maven 3.1.0-alpha-1 |
| 7.X.X | Maven 3.2.5 |
| 8.X.X | Maven 3.2.5 |
Flipping the table to maven:
Please note that in theory maven 4.X should support all maven 3 plugins.
The plugin was first shipped with maven 3 support in version v2.1.14 (requiring maven version 3.1.1).
Hence the v2.1.14 should be the first supported version.
Only starting with 6.X.X this plugin was acually tested with 4.0.0-alpha-5,
but some releases might not work since Maven 4 announced that plugins require Maven 3.2.5 or later
which would only be the case for plugin versions 7.0.0 or later.
| Maven Version | Plugin Version | Notes |
|---------------|----------------:|:--------------------------------------------------:|
| Maven 3.X | any | The plugin requires at least a maven 3.1.0-alpha-1 |
| Maven 4.X | from v2.1.14 | |
Plugin compatibility with EOL Maven version
-----------------------------
End of life (EOL) Maven versions are no longer supported by Maven, nor this plugin.
The following information is made available for reference.
| Maven Version | Plugin Version | Notes |
| --------------------------- | ---------------:|:---------------------------------------------------------------------------------------------------------------:|
| Maven 2.0.11 | up to 2.2.6 | Maven 2 is EOL, git-commit-id-plugin:1.0 doesn't work -- requires maven version 2.2.1 |
| Maven 2.2.1 | up to 2.2.6 | Maven 2 is EOL |
| Maven 3.0.X | up to 4.0.5 | git-commit-id-plugin:2.1.14, 2.1.15, 2.2.0, 2.2.1, 2.2.3 doesn't work -- requires maven version 3.1.1 |
| Maven 3.0.X | up to 4.0.5 | For git-commit-id-plugin 2.2.4 or higher: works, but failed to load class "org.slf4j.impl.StaticLoggerBinder" |
| Maven 3.1.0 | any | git-commit-id-plugin:2.1.14, 2.1.15, 2.2.0, 2.2.1, 2.2.3 doesn't work -- requires maven version 3.1.1 |
| Maven 3.3.1 | any | git-commit-id-plugin:2.1.14 doesn't work |
| Maven 3.3.3 | any | git-commit-id-plugin:2.1.14 doesn't work |
Note:
As an example -- this table should be read as: For `Maven 3.1.0` `any` Plugin Version should work, besides the ones listed in the `Notes` have the limitations listed.
Getting SNAPSHOT versions of the plugin
---------------------------------------
If you really want to use **snapshots**, here's the repository they are deployed to.
But I highly recommend using only stable versions, from Maven Central... :-)
```xml
<pluginRepositories>
<pluginRepository>
<id>sonatype-snapshots</id>
<name>Sonatype Snapshots</name>
<url>https://s01.oss.sonatype.org/content/repositories/snapshots/</url>
</pluginRepository>
</pluginRepositories>
```
Older Snapshots (prior version 5.X) are available via `<url>https://oss.sonatype.org/content/repositories/snapshots/</url>`.
If you just would like to see what the plugin can do, you can clone the repository and run
```
mvn clean install -Dmaven.test.skip=true && mvn clean package -Pdemo -Dmaven.test.skip=true
```
Maintainers
===========
This project is currently maintained thanks to: @ktoso (founder), @TheSnoozer
Notable contributions
=====================
I'd like to give a big thanks to some of these folks, for their suggestions and / or pull requests that helped make this plugin as popular as it is today:
* @mostr - for bugfixes and a framework to do integration testing,
* @fredcooke - for consistent feedback and suggestions,
* @MrOnion - for a small yet fast bugfix,
* @cardil and @TheSnoozer - for helping with getting the native git support shipped,
* all the other contributors (as of writing 50) which can be on the [contributors tab](https://github.com/git-commit-id/git-commit-id-maven-plugin/graphs/contributors) - thanks guys,
* ... many others - thank you for your contributions,
* ... you! - for using the plugin :-)
Notable happy users
===================
* [neo4j](https://neo4j.com/) – graph database
* [FoundationdDB](https://www.foundationdb.org/) – another open source database
* [Spring Boot](https://docs.spring.io/spring-boot/docs/current/reference/htmlsingle/#using-boot-maven) – yes, the upstream Spring project is using us
* Akamai, Sabre, EasyDITA, and many many others,
* many others I don't know of.
License
=======
<img style="float:right; padding:3px; " src="https://github.com/git-commit-id/git-commit-id-maven-plugin/raw/master/lgplv3-147x51.png" alt="GNU LGPL v3"/>
I'm releasing this plugin under the **GNU Lesser General Public License 3.0**.
You're free to use it as you wish, the full license text is attached in the LICENSE file.
Feature requests
================
The best way to ask for features / improvements is [via the Issues section on GitHub - it's better than email](https://github.com/git-commit-id/git-commit-id-maven-plugin/issues) because I won't loose when I have a "million emails inbox" day,
and maybe someone else has some idea or would like to upvote your issue.
That's all folks! **Happy hacking!**
| 0 |
BroadleafCommerce/BroadleafCommerce | Broadleaf Commerce CE - an eCommerce framework based on Java and Spring | java spring spring-boot spring-mvc spring-security | ## Broadleaf Commerce Community Edition (CE)
Broadleaf Commerce CE is an e-commerce framework written entirely in Java and leveraging the Spring framework. It is targeted at facilitating the development of enterprise-class, commerce-driven sites by providing a robust data model, services and specialized tooling that take care of most of the "heavy lifting" work. To accomplish this goal, we have developed the core platform based on the key feature sets required by world-class online retailers. We've also taken extra steps to guarantee interoperability with today's enterprise by utilizing standards wherever possible and incorporating best-of-breed, open-source software libraries. Broadleaf CE and EE are architected as a traditional unified codebase that share a core dependency across a `site` and `admin` deployment. If you are looking for a microservices based architecture, check out our [Microservices Edition](https://developer.broadleafcommerce.com/)
## Editions
There are three editions of Broadleaf:
- Broadleaf Commerce Community Edition (CE) - open source under a dual licence format with both fair use and commercial restrictions. See dual license below for more details.
- Broadleaf Commerce Enterprise Edition (EE) - source available under a commercial license. Built on top of Broadleaf's open core platform, this edition includes additional features and support for B2C, B2B, Multi-Tenant, and Marketplace use cases.
- Broadleaf Commerce Microservices Edition - source available under a commercial license. This next generation platform provides a [microservice enablement framework](https://developer.broadleafcommerce.com/architecture) consisting of headless commerce APIs that support many enterprise use cases. Please [contact us](http://broadleafcommerce.com/contact) or sign up for a [Developer Evaluation](https://www.broadleafcommerce.com/developer-evaluation) for more information.
## CE Dual License
Broadleaf Commerce core is released under a dual license format. It may be used under the terms of the Fair Use License 1.0 (http://license.broadleafcommerce.org/fair_use_license-1.0.txt) unless the restrictions on use therein are violated and require payment to Broadleaf, in which case the Broadleaf End User License Agreement (EULA), Version 1.1 (http://license.broadleafcommerce.org/commercial_license-1.1.txt) shall apply. Alternatively, the Commercial License may be replaced with a mutually agreed upon license between you and Broadleaf Commerce.
## Getting Started
Check out our [Getting Started guide](https://www.broadleafcommerce.com/docs/core/current/tutorials/getting-started-tutorials) to quickly kick off your Broadleaf-enabled website.
## Key Features and Technologies
### Spring Framework
Spring is the enterprise Java platform on which BroadleafCommerce is based. It provides numerous features, including dependency injection and transaction control.
### Security
Spring Security provides a robust security framework for controlling authentication and authorization at both the code and page level and is utilized by BroadleafCommerce for access control.
### Persistence
JPA and Hibernate represent the BroadleafCommerce ORM infrastructure for
controlling persistence of our rich domain model.
### Search
Flexible domain search capabilities in BroadleafCommerce are provided through integration
with Solr.
### Task Scheduling
Scheduling of repetitive tasks in BroadleafCommerce is offered through the
Quartz job scheduling system.
### Email
Email support is provided throughout the BroadleafCommerce framework in either synchronous
or asynchronous (JMS) modes. Email presentation customization is achieved via Thymeleaf templates.
### Modular Design
Important e-commerce touchpoints are embodied in the concept of BroadleafCommerce
"Modules". A module can provide interaction with a credit card processor, or even a shipping provider.
Any number of custom modules may be developed and utilized with BroadleafCommerce.
### Configurable Workflows
Key areas in the e-commerce lifecycle are represented as configurable
workflows. Implementors have full control over the keys steps in pricing and checkout, allowing
manipulation of module ordering, overriding existing module behavior and custom module execution.
Composite workflows are also supported to achieve more exotic, nested behavior.
### Extendible Design
BroadleafCommerce is designed from the ground-up with extensibility in mind.
Almost every aspect of BroadleafCommerce can be overridden, added to or otherwise modified to enhance
or change the default behavior to best fit your needs. This includes all of our services, data access
objects and entities. Please refer to the extensibility section of our documentation.
### Configuration Merging
As an extra bonus to our extensibility model, we offer a custom merge facility
for Spring configuration files. We minimize the BroadleafCommerce configuration semantics that an
implementer must be aware of, allowing our users to focus on their own configuration particulars.
BroadleafCommerce will intelligently merge its own configuration information with that provided by
the implementer at runtime.
### Presentation Layer Support
BroadleafCommerce also includes a number of pre-written Spring MVC
controllers that help to speed development of the presentation layer of your own BroadleafCommerce-driven
site.
### QOS
BroadleafCommerce also provides quality of service monitoring for modules (both custom and
default modules) and provides support for several QOS handlers out-of-the-box: logging and email.
Additional, custom QOS handlers may be added through our open API.
### Promotion System
BroadleafCommerce includes a highly-configurable system for including your pricing
promotions. We provide several standard levels at which promotions may be applied: Order level, Order
Item level and Fulfillment Group level. In addition, your promotion business rules are represented in
a flexible and standardized way using the MVEL expression language.
### PCI Considerations
We have taken measures in the construction and design of BroadleafCommerce to
help you achieve PCI compliance, should you decide to store and use sensitive customer financial
account information. Payment account information is referenced separately, allowing you to segregate
confidential data onto a separate, secure database platform. API methods have been added to allow
inclusion of any PCI compliant encryption scheme. Also, verbose logging is included to track payment
interaction history.
### Admin Platform
BroadleafCommerce includes a wholely extendible administrative application built with Spring MVC. The admin application also provides an easy-to-use interface
for catalog, order and customer functions and provides a robust, rule-driven environment for creating
and managing discount promotions.
### Admin Customization
BroadleafCommerce provides a robust set of admin presentation annotations that allow configuration of domain
class display and persistence semantics without touching any admin code. This provides an easy-to-consume approach
for introducing entity extensions and additional fields into the admin forms so that your business users can immediately
start to benefit. We also provide a full annotation or xml-based approach for overriding the admin config declared
inside BroadleafCommerce so that you can have an impact on our defaults. And for more advanced customizations, our admin
platform is based on Spring MVC, so your Spring knowledge will translate here as well when it comes to adding additional
controllers, and the like.
### Content Management
BroadleafCommerce includes a robust content management system for creating and
managing static pages and content. We also include a powerful content targeting feature that allows
business users to dynamically drive the most appropriate content to users.
Please [contact us](http://broadleafcommerce.com/contact) for information.
| 0 |
marytts/marytts | MARY TTS -- an open-source, multilingual text-to-speech synthesis system written in pure java | java speech-synthesis text-to-speech tts | [](https://github.com/marytts/marytts/actions/workflows/main.yml)
# MaryTTS
This is the source code repository for the multilingual open-source MARY text-to-speech platform (MaryTTS).
MaryTTS is a client-server system written in pure Java, so it runs on many platforms.
**For a downloadable package ready for use, see [the releases page](https://github.com/marytts/marytts/releases).**
Older documentation can also be found at https://github.com/marytts/marytts-wiki, http://mary.dfki.de and https://mary.opendfki.de.
This README is part of the the MaryTTS source code repository.
It contains information about compiling and developing the MaryTTS sources.
The code comes under the Lesser General Public License LGPL version 3 -- see LICENSE.md for details.
## Running MaryTTS
Run `./gradlew run` (or `gradlew.bat run` on Windows) to start a MaryTTS server.
Then access it at http://localhost:59125 using your web browser.
If you want to start a MaryTTS on a different address and port, you can use the following options:
```sh
./gradlew run -Dsocket.port=5920 -Dsocket.addr=0.0.0.0 --info
```
where 5920 is the new port and 0.0.0.0 the new address. In case of the address being 0.0.0.0, all the interfaces will be listened.
By using the option `--info`, you set the logger of `gradle` *AND* MaryTTS at the level INFO. By using `--debug`, you set the level to DEBUG.
It is also possible to set the MaryTTS logger level to `INFO` or `DEBUG` by defining the system variable `log4j.logger.marytts`.
## Downloading and installing voices
Run `./gradlew runInstallerGui` to start an installer GUI to download and install more voices.
A running MaryTTS server needs to be restarted before the new voices can be used.
## Building MaryTTS
Run `./gradlew build`.
This will compile and test all modules, and create the output for each under `build/`.
Note that previously, MaryTTS v5.x was built with Maven. Please refer to the [**5.x branch**](https://github.com/marytts/marytts/tree/5.x).
## Packaging MaryTTS
Run `./gradlew distZip` or `./gradlew distTar` to build a distribution package under `build/distributions`.
You can also "install" an unpacked distribution directly into `build/install` by running `./gradlew installDist`.
The distribution contains all the files required to run a standalone MaryTTS server instance, or to download and install more voices.
The scripts to run the server or installer GUI can be found inside the distribution in the `bin/` directory.
## Using MaryTTS in your own Java projects
The easiest way to use MaryTTS in your own Java projects is to declare a dependency on a relevant MaryTTS artifact, such as the default US English HSMM voice:
### Maven
Add to your `pom.xml`:
```xml
<repositories>
<repository>
<url>https://mlt.jfrog.io/artifactory/mlt-mvn-releases-local</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>de.dfki.mary</groupId>
<artifactId>voice-cmu-slt-hsmm</artifactId>
<version>5.2.1</version>
<exclusions>
<exclusion>
<groupId>com.twmacinta</groupId>
<artifactId>fast-md5</artifactId>
</exclusion>
<exclusion>
<groupId>gov.nist.math</groupId>
<artifactId>Jampack</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
```
### Gradle
Add to your `build.gradle`:
```groovy
repositories {
mavenCentral()
exclusiveContent {
forRepository {
maven {
url 'https://mlt.jfrog.io/artifactory/mlt-mvn-releases-local'
}
}
filter {
includeGroup 'de.dfki.lt.jtok'
}
}
}
dependencies {
implementation group: 'de.dfki.mary', name: 'voice-cmu-slt-hsmm', version: '5.2.1', {
exclude group: 'com.twmacinta', module: 'fast-md5'
exclude group: 'gov.nist.math', module: 'Jampack'
}
}
```
## Synthesizing speech
Text to wav basic examples are proposed in this repository
- Maven: https://github.com/marytts/marytts-txt2wav/tree/maven
- Gradle: https://github.com/marytts/marytts-txt2wav/tree/gradle
## Using MaryTTS for other programming languages
If you want to use MaryTTS for other programming languages (like python for example), you need to achieve 3 steps
1. compiling marytts
2. starting the server
3. query synthesis on the server
### Synthesize speech using the server
Synthesizing speech, using the server, is pretty easy.
You need to generate proper HTTP queries and deal with the associated HTTP responses.
Examples are proposed :
- python 3: https://github.com/marytts/marytts-txt2wav/tree/python
- shell: https://github.com/marytts/marytts-txt2wav/tree/sh
## Extra documentation
### Server as service (Linux specific)
An example of how to define marytts server as service is proposed [here](./src/main/dist/misc/marytts.server).
### User dictionaries
You can extend the dictionaries by adding a user dictionary. The documentation of how to do it is [here](./src/main/dist/user-dictionaries/README.md).
## Contributing
The recommended workflow for making contributions to the MaryTTS source code is to follow the GitHub model:
1. fork the MaryTTS repository into your own profile on GitHub, by navigating to https://github.com/marytts/marytts and clicking "fork" (of course you need a GitHub account);
2. use the `git clone`, `commit`, and `push` commands to make modifications on your own marytts repository;
in this process, make sure to `git pull upstream master` regularly to stay in sync with latest developments on the master repo;
3. when you think a reusable contribution is ready, open a "pull request" on GitHub to allow for easy merging into the master repository.
Have a look at the [GitHub documentation](http://help.github.com/) for further details.
### IDE configuration
Wiki pages are available to help you to configure your IDE to develop MaryTTS.
The following IDEs have been tested and documented:
- IntelliJ IDEA
- Eclipse: https://github.com/marytts/marytts/wiki/Eclipse
| 0 |
houbb/sensitive-word | 👮♂️The sensitive word tool for java.(敏感词/违禁词/违法词/脏词。基于 DFA 算法实现的高性能 java 敏感词过滤工具框架。请勿发布涉及政治、广告、营销、翻墙、违反国家法律法规等内容。高性能敏感词检测过滤组件,附带繁体简体互换,支持全角半角互换,汉字转拼音,模糊搜索等功能。) | dfa dirty-word nlp sensitive sensitive-word sensitive-word-filter trie-tree | # sensitive-word
[sensitive-word](https://github.com/houbb/sensitive-word) 基于 DFA 算法实现的高性能敏感词工具。
[](http://mvnrepository.com/artifact/com.github.houbb/sensitive-word)
[](https://github.com/houbb/sensitive-word)
[](https://github.com/houbb/sensitive-word/blob/master/LICENSE.txt)
> [在线体验](https://houbb.github.io/opensource/sensitive-word)
如果有一些疑难杂症,可以加入:[技术交流群](https://mp.weixin.qq.com/s/rkSvXxiiLGjl3S-ZOZCr0Q)
[sensitive-word-admin](https://github.com/houbb/sensitive-word-admin) 是对应的控台的应用,目前功能处于初期开发中,MVP 版本可用。
## 创作目的
实现一款好用敏感词工具。
基于 DFA 算法实现,目前敏感词库内容收录 6W+(源文件 18W+,经过一次删减)。
后期将进行持续优化和补充敏感词库,并进一步提升算法的性能。
希望可以细化敏感词的分类,感觉工作量比较大,暂时没有进行。
## 特性
- 6W+ 词库,且不断优化更新
- 基于 fluent-api 实现,使用优雅简洁
- [基于 DFA 算法,性能为 7W+ QPS,应用无感](https://github.com/houbb/sensitive-word#benchmark)
- [支持敏感词的判断、返回、脱敏等常见操作](https://github.com/houbb/sensitive-word#%E6%A0%B8%E5%BF%83%E6%96%B9%E6%B3%95)
- [支持常见的格式转换](https://github.com/houbb/sensitive-word#%E6%9B%B4%E5%A4%9A%E7%89%B9%E6%80%A7)
全角半角互换、英文大小写互换、数字常见形式的互换、中文繁简体互换、英文常见形式的互换、忽略重复词等
- [支持敏感词检测、邮箱检测、数字检测、网址检测等](https://github.com/houbb/sensitive-word#%E6%9B%B4%E5%A4%9A%E6%A3%80%E6%B5%8B%E7%AD%96%E7%95%A5)
- [支持自定义替换策略](https://github.com/houbb/sensitive-word#%E8%87%AA%E5%AE%9A%E4%B9%89%E6%9B%BF%E6%8D%A2%E7%AD%96%E7%95%A5)
- [支持用户自定义敏感词和白名单](https://github.com/houbb/sensitive-word#%E9%85%8D%E7%BD%AE%E4%BD%BF%E7%94%A8)
- [支持数据的数据动态更新(用户自定义),实时生效](https://github.com/houbb/sensitive-word#%E5%8A%A8%E6%80%81%E5%8A%A0%E8%BD%BD%E7%94%A8%E6%88%B7%E8%87%AA%E5%AE%9A%E4%B9%89)
- [支持敏感词的标签接口](https://github.com/houbb/sensitive-word#%E6%95%8F%E6%84%9F%E8%AF%8D%E6%A0%87%E7%AD%BE)
- [支持跳过一些特殊字符,让匹配更灵活](https://github.com/houbb/sensitive-word#%E5%BF%BD%E7%95%A5%E5%AD%97%E7%AC%A6)
## 变更日志
[CHANGE_LOG.md](https://github.com/houbb/sensitive-word/blob/master/CHANGE_LOG.md)
V0.14.0: raw 添加敏感词类别。
## 更多资料
### 敏感词控台
有时候敏感词有一个控台,配置起来会更加灵活方便。
> [java 如何实现开箱即用的敏感词控台服务?](https://mp.weixin.qq.com/s/rQo75cfMU_OEbTJa0JGMGg)
### 敏感词标签文件
梳理了大量的敏感词标签文件,可以让我们的敏感词更加方便。
这两个资料阅读可在下方文章获取:
> [v0.11.0-敏感词新特性及对应标签文件](https://mp.weixin.qq.com/s/m40ZnR6YF6WgPrArUSZ_0g)
# 快速开始
## 准备
- JDK1.7+
- Maven 3.x+
## Maven 引入
```xml
<dependency>
<groupId>com.github.houbb</groupId>
<artifactId>sensitive-word</artifactId>
<version>0.14.0</version>
</dependency>
```
## 核心方法
`SensitiveWordHelper` 作为敏感词的工具类,核心方法如下:
| 方法 | 参数 | 返回值 | 说明 |
|:---------------------------------------|:-------------------------|:-------|:-------------|
| contains(String) | 待验证的字符串 | 布尔值 | 验证字符串是否包含敏感词 |
| replace(String, ISensitiveWordReplace) | 使用指定的替换策略替换敏感词 | 字符串 | 返回脱敏后的字符串 |
| replace(String, char) | 使用指定的 char 替换敏感词 | 字符串 | 返回脱敏后的字符串 |
| replace(String) | 使用 `*` 替换敏感词 | 字符串 | 返回脱敏后的字符串 |
| findAll(String) | 待验证的字符串 | 字符串列表 | 返回字符串中所有敏感词 |
| findFirst(String) | 待验证的字符串 | 字符串 | 返回字符串中第一个敏感词 |
| findAll(String, IWordResultHandler) | IWordResultHandler 结果处理类 | 字符串列表 | 返回字符串中所有敏感词 |
| findFirst(String, IWordResultHandler) | IWordResultHandler 结果处理类 | 字符串 | 返回字符串中第一个敏感词 |
| tags(String) | 获取敏感词的标签 | 敏感词字符串 | 返回敏感词的标签列表 |
### 判断是否包含敏感词
```java
final String text = "五星红旗迎风飘扬,毛主席的画像屹立在天安门前。";
Assert.assertTrue(SensitiveWordHelper.contains(text));
```
### 返回第一个敏感词
```java
final String text = "五星红旗迎风飘扬,毛主席的画像屹立在天安门前。";
String word = SensitiveWordHelper.findFirst(text);
Assert.assertEquals("五星红旗", word);
```
SensitiveWordHelper.findFirst(text) 等价于:
```java
String word = SensitiveWordHelper.findFirst(text, WordResultHandlers.word());
```
### 返回所有敏感词
```java
final String text = "五星红旗迎风飘扬,毛主席的画像屹立在天安门前。";
List<String> wordList = SensitiveWordHelper.findAll(text);
Assert.assertEquals("[五星红旗, 毛主席, 天安门]", wordList.toString());
```
返回所有敏感词用法上类似于 SensitiveWordHelper.findFirst(),同样也支持指定结果处理类。
SensitiveWordHelper.findAll(text) 等价于:
```java
List<String> wordList = SensitiveWordHelper.findAll(text, WordResultHandlers.word());
```
WordResultHandlers.raw() 可以保留对应的下标信息、类别信息:
```java
final String text = "骂人:你他妈; 邮箱:123@qq.com; mobile: 13088889999; 网址:https://www.baidu.com";
List<IWordResult> wordList3 = SensitiveWordHelper.findAll(text, WordResultHandlers.raw());
Assert.assertEquals("[WordResult{startIndex=3, endIndex=6, type='WORD'}, WordResult{startIndex=11, endIndex=21, type='EMAIL'}, WordResult{startIndex=31, endIndex=42, type='NUM'}, WordResult{startIndex=55, endIndex=68, type='URL'}]", wordList3.toString());
```
### 默认的替换策略
```java
final String text = "五星红旗迎风飘扬,毛主席的画像屹立在天安门前。";
String result = SensitiveWordHelper.replace(text);
Assert.assertEquals("****迎风飘扬,***的画像屹立在***前。", result);
```
### 指定替换的内容
```java
final String text = "五星红旗迎风飘扬,毛主席的画像屹立在天安门前。";
String result = SensitiveWordHelper.replace(text, '0');
Assert.assertEquals("0000迎风飘扬,000的画像屹立在000前。", result);
```
### 自定义替换策略
V0.2.0 支持该特性。
场景说明:有时候我们希望不同的敏感词有不同的替换结果。比如【游戏】替换为【电子竞技】,【失业】替换为【灵活就业】。
诚然,提前使用字符串的正则替换也可以,不过性能一般。
使用例子:
```java
/**
* 自定替换策略
* @since 0.2.0
*/
@Test
public void defineReplaceTest() {
final String text = "五星红旗迎风飘扬,毛主席的画像屹立在天安门前。";
ISensitiveWordReplace replace = new MySensitiveWordReplace();
String result = SensitiveWordHelper.replace(text, replace);
Assert.assertEquals("国家旗帜迎风飘扬,教员的画像屹立在***前。", result);
}
```
其中 `MySensitiveWordReplace` 是我们自定义的替换策略,实现如下:
```java
public class MyWordReplace implements IWordReplace {
@Override
public void replace(StringBuilder stringBuilder, final char[] rawChars, IWordResult wordResult, IWordContext wordContext) {
String sensitiveWord = InnerWordCharUtils.getString(rawChars, wordResult);
// 自定义不同的敏感词替换策略,可以从数据库等地方读取
if("五星红旗".equals(sensitiveWord)) {
stringBuilder.append("国家旗帜");
} else if("毛主席".equals(sensitiveWord)) {
stringBuilder.append("教员");
} else {
// 其他默认使用 * 代替
int wordLength = wordResult.endIndex() - wordResult.startIndex();
for(int i = 0; i < wordLength; i++) {
stringBuilder.append('*');
}
}
}
}
```
我们针对其中的部分词做固定映射处理,其他的默认转换为 `*`。
## IWordResultHandler 结果处理类
IWordResultHandler 可以对敏感词的结果进行处理,允许用户自定义。
内置实现见 `WordResultHandlers` 工具类:
- WordResultHandlers.word()
只保留敏感词单词本身。
- WordResultHandlers.raw()
保留敏感词相关信息,包含敏感词的开始和结束下标。
- WordResultHandlers.wordTags()
同时保留单词,和对应的词标签信息。
### 使用实例
所有测试案例参见 [SensitiveWordHelperTest](https://github.com/houbb/sensitive-word/blob/master/src/test/java/com/github/houbb/sensitive/word/core/SensitiveWordHelperTest.java)
1)基本例子
```java
final String text = "五星红旗迎风飘扬,毛主席的画像屹立在天安门前。";
List<String> wordList = SensitiveWordHelper.findAll(text);
Assert.assertEquals("[五星红旗, 毛主席, 天安门]", wordList.toString());
List<String> wordList2 = SensitiveWordHelper.findAll(text, WordResultHandlers.word());
Assert.assertEquals("[五星红旗, 毛主席, 天安门]", wordList2.toString());
List<IWordResult> wordList3 = SensitiveWordHelper.findAll(text, WordResultHandlers.raw());
Assert.assertEquals("[WordResult{startIndex=0, endIndex=4}, WordResult{startIndex=9, endIndex=12}, WordResult{startIndex=18, endIndex=21}]", wordList3.toString());
```
2) wordTags 例子
我们在 `dict_tag_test.txt` 文件中指定对应词的标签信息。
```java
final String text = "五星红旗迎风飘扬,毛主席的画像屹立在天安门前。";
// 默认敏感词标签为空
List<WordTagsDto> wordList1 = SensitiveWordHelper.findAll(text, WordResultHandlers.wordTags());
Assert.assertEquals("[WordTagsDto{word='五星红旗', tags=[]}, WordTagsDto{word='毛主席', tags=[]}, WordTagsDto{word='天安门', tags=[]}]", wordList1.toString());
List<WordTagsDto> wordList2 = SensitiveWordBs.newInstance()
.wordTag(WordTags.file("dict_tag_test.txt"))
.init()
.findAll(text, WordResultHandlers.wordTags());
Assert.assertEquals("[WordTagsDto{word='五星红旗', tags=[政治, 国家]}, WordTagsDto{word='毛主席', tags=[政治, 伟人, 国家]}, WordTagsDto{word='天安门', tags=[政治, 国家, 地址]}]", wordList2.toString());
```
# 更多特性
后续的诸多特性,主要是针对各种针对各种情况的处理,尽可能的提升敏感词命中率。
这是一场漫长的攻防之战。
## 样式处理
### 忽略大小写
```java
final String text = "fuCK the bad words.";
String word = SensitiveWordHelper.findFirst(text);
Assert.assertEquals("fuCK", word);
```
### 忽略半角圆角
```java
final String text = "fuck the bad words.";
String word = SensitiveWordHelper.findFirst(text);
Assert.assertEquals("fuck", word);
```
### 忽略数字的写法
这里实现了数字常见形式的转换。
```java
final String text = "这个是我的微信:9⓿二肆⁹₈③⑸⒋➃㈤㊄";
List<String> wordList = SensitiveWordHelper.findAll(text);
Assert.assertEquals("[9⓿二肆⁹₈③⑸⒋➃㈤㊄]", wordList.toString());
```
### 忽略繁简体
```java
final String text = "我爱我的祖国和五星紅旗。";
List<String> wordList = SensitiveWordHelper.findAll(text);
Assert.assertEquals("[五星紅旗]", wordList.toString());
```
### 忽略英文的书写格式
```java
final String text = "Ⓕⓤc⒦ the bad words";
List<String> wordList = SensitiveWordHelper.findAll(text);
Assert.assertEquals("[Ⓕⓤc⒦]", wordList.toString());
```
### 忽略重复词
```java
final String text = "ⒻⒻⒻfⓤuⓤ⒰cⓒ⒦ the bad words";
List<String> wordList = SensitiveWordBs.newInstance()
.ignoreRepeat(true)
.init()
.findAll(text);
Assert.assertEquals("[ⒻⒻⒻfⓤuⓤ⒰cⓒ⒦]", wordList.toString());
```
## 更多检测策略
### 邮箱检测
```java
final String text = "楼主好人,邮箱 sensitiveword@xx.com";
List<String> wordList = SensitiveWordHelper.findAll(text);
Assert.assertEquals("[sensitiveword@xx.com]", wordList.toString());
```
### 连续数字检测
一般用于过滤手机号/QQ等广告信息。
V0.2.1 之后,支持通过 `numCheckLen(长度)` 自定义检测的长度。
```java
final String text = "你懂得:12345678";
// 默认检测 8 位
List<String> wordList = SensitiveWordBs.newInstance().init().findAll(text);
Assert.assertEquals("[12345678]", wordList.toString());
// 指定数字的长度,避免误杀
List<String> wordList2 = SensitiveWordBs.newInstance()
.numCheckLen(9)
.init()
.findAll(text);
Assert.assertEquals("[]", wordList2.toString());
```
### 网址检测
用于过滤常见的网址信息。
```java
final String text = "点击链接 www.baidu.com查看答案";
List<String> wordList = SensitiveWordBs.newInstance().init().findAll(text);
Assert.assertEquals("[链接, www.baidu.com]", wordList.toString());
Assert.assertEquals("点击** *************查看答案", SensitiveWordBs
.newInstance()
.init()
.replace(text));
```
# 引导类特性配置
## 说明
上面的特性默认都是开启的,有时业务需要灵活定义相关的配置特性。
所以 v0.0.14 开放了属性配置。
## 配置方法
为了让使用更加优雅,统一使用 fluent-api 的方式定义。
用户可以使用 `SensitiveWordBs` 进行如下定义:
```java
SensitiveWordBs wordBs = SensitiveWordBs.newInstance()
.ignoreCase(true)
.ignoreWidth(true)
.ignoreNumStyle(true)
.ignoreChineseStyle(true)
.ignoreEnglishStyle(true)
.ignoreRepeat(false)
.enableNumCheck(true)
.enableEmailCheck(true)
.enableUrlCheck(true)
.enableWordCheck(true)
.numCheckLen(8)
.wordTag(WordTags.none())
.charIgnore(SensitiveWordCharIgnores.defaults())
.wordResultCondition(WordResultConditions.alwaysTrue())
.init();
final String text = "五星红旗迎风飘扬,毛主席的画像屹立在天安门前。";
Assert.assertTrue(wordBs.contains(text));
```
## 配置说明
其中各项配置的说明如下:
| 序号 | 方法 | 说明 | 默认值 |
|:---|:---------------------|:-----------------------------|:------|
| 1 | ignoreCase | 忽略大小写 | true |
| 2 | ignoreWidth | 忽略半角圆角 | true |
| 3 | ignoreNumStyle | 忽略数字的写法 | true |
| 4 | ignoreChineseStyle | 忽略中文的书写格式 | true |
| 5 | ignoreEnglishStyle | 忽略英文的书写格式 | true |
| 6 | ignoreRepeat | 忽略重复词 | false |
| 7 | enableNumCheck | 是否启用数字检测。 | true |
| 8 | enableEmailCheck | 是有启用邮箱检测 | true |
| 9 | enableUrlCheck | 是否启用链接检测 | true |
| 10 | enableWordCheck | 是否启用敏感单词检测 | true |
| 11 | numCheckLen | 数字检测,自定义指定长度。 | 8 |
| 12 | wordTag | 词对应的标签 | none |
| 13 | charIgnore | 忽略的字符 | none |
| 14 | wordResultCondition | 针对匹配的敏感词额外加工,比如可以限制英文单词必须全匹配 | 恒为真 |
# wordResultCondition-针对匹配词进一步判断
## 说明
支持版本:v0.13.0
有时候我们可能希望对匹配的敏感词进一步限制,比如虽然我们定义了【av】作为敏感词,但是不希望【have】被匹配。
就可以自定义实现 wordResultCondition 接口,实现自己的策略。
系统内置的策略在 `WordResultConditions#alwaysTrue()` 恒为真,`WordResultConditions#englishWordMatch()` 则要求英文必须全词匹配。
## 入门例子
原始的默认情况:
```java
final String text = "I have a nice day。";
List<String> wordList = SensitiveWordBs.newInstance()
.wordDeny(new IWordDeny() {
@Override
public List<String> deny() {
return Collections.singletonList("av");
}
})
.wordResultCondition(WordResultConditions.alwaysTrue())
.init()
.findAll(text);
Assert.assertEquals("[av]", wordList.toString());
```
我们可以指定为英文必须全词匹配。
```java
final String text = "I have a nice day。";
List<String> wordList = SensitiveWordBs.newInstance()
.wordDeny(new IWordDeny() {
@Override
public List<String> deny() {
return Collections.singletonList("av");
}
})
.wordResultCondition(WordResultConditions.englishWordMatch())
.init()
.findAll(text);
Assert.assertEquals("[]", wordList.toString());
```
当然可以根据需要实现更加复杂的策略。
# 忽略字符
## 说明
我们的敏感词一般都是比较连续的,比如【傻帽】
那就有大聪明发现,可以在中间加一些字符,比如【傻!@#$帽】跳过检测,但是骂人等攻击力不减。
那么,如何应对这些类似的场景呢?
我们可以指定特殊字符的跳过集合,忽略掉这些无意义的字符即可。
v0.11.0 开始支持
## 例子
其中 charIgnore 对应的字符策略,用户可以自行灵活定义。
```java
final String text = "傻@冒,狗+东西";
//默认因为有特殊字符分割,无法识别
List<String> wordList = SensitiveWordBs.newInstance().init().findAll(text);
Assert.assertEquals("[]", wordList.toString());
// 指定忽略的字符策略,可自行实现。
List<String> wordList2 = SensitiveWordBs.newInstance()
.charIgnore(SensitiveWordCharIgnores.specialChars())
.init()
.findAll(text);
Assert.assertEquals("[傻@冒, 狗+东西]", wordList2.toString());
```
# 敏感词标签
## 说明
有时候我们希望对敏感词加一个分类标签:比如社情、暴/力等等。
这样后续可以按照标签等进行更多特性操作,比如只处理某一类的标签。
支持版本:v0.10.0
## 入门例子
### 接口
这里只是一个抽象的接口,用户可以自行定义实现。比如从数据库查询等。
```java
public interface IWordTag {
/**
* 查询标签列表
* @param word 脏词
* @return 结果
*/
Set<String> getTag(String word);
}
```
### 配置文件
我们可以自定义 dict 标签文件,通过 WordTags.file() 创建一个 WordTag 实现。
- dict_tag_test.txt
```
五星红旗 政治,国家
```
格式如下:
```
敏感词 tag1,tag2
```
### 实现
具体的效果如下,在引导类设置一下即可。
默认的 wordTag 是空的。
```java
String filePath = "dict_tag_test.txt";
IWordTag wordTag = WordTags.file(filePath);
SensitiveWordBs sensitiveWordBs = SensitiveWordBs.newInstance()
.wordTag(wordTag)
.init();
Assert.assertEquals("[政治, 国家]", sensitiveWordBs.tags("五星红旗").toString());;
```
后续会考虑引入一个内置的标签文件策略。
# 动态加载(用户自定义)
## 情景说明
有时候我们希望将敏感词的加载设计成动态的,比如控台修改,然后可以实时生效。
v0.0.13 支持了这种特性。
## 接口说明
为了实现这个特性,并且兼容以前的功能,我们定义了两个接口。
### IWordDeny
接口如下,可以自定义自己的实现。
返回的列表,表示这个词是一个敏感词。
```java
/**
* 拒绝出现的数据-返回的内容被当做是敏感词
* @author binbin.hou
* @since 0.0.13
*/
public interface IWordDeny {
/**
* 获取结果
* @return 结果
* @since 0.0.13
*/
List<String> deny();
}
```
比如:
```java
public class MyWordDeny implements IWordDeny {
@Override
public List<String> deny() {
return Arrays.asList("我的自定义敏感词");
}
}
```
### IWordAllow
接口如下,可以自定义自己的实现。
返回的列表,表示这个词不是一个敏感词。
```java
/**
* 允许的内容-返回的内容不被当做敏感词
* @author binbin.hou
* @since 0.0.13
*/
public interface IWordAllow {
/**
* 获取结果
* @return 结果
* @since 0.0.13
*/
List<String> allow();
}
```
如:
```java
public class MyWordAllow implements IWordAllow {
@Override
public List<String> allow() {
return Arrays.asList("五星红旗");
}
}
```
## 配置使用
**接口自定义之后,当然需要指定才能生效。**
为了让使用更加优雅,我们设计了引导类 `SensitiveWordBs`。
可以通过 wordDeny() 指定敏感词,wordAllow() 指定非敏感词,通过 init() 初始化敏感词字典。
### 系统的默认配置
```java
SensitiveWordBs wordBs = SensitiveWordBs.newInstance()
.wordDeny(WordDenys.defaults())
.wordAllow(WordAllows.defaults())
.init();
final String text = "五星红旗迎风飘扬,毛主席的画像屹立在天安门前。";
Assert.assertTrue(wordBs.contains(text));
```
备注:init() 对于敏感词 DFA 的构建是比较耗时的,一般建议在应用初始化的时候**只初始化一次**。而不是重复初始化!
### 指定自己的实现
我们可以测试一下自定义的实现,如下:
```java
String text = "这是一个测试,我的自定义敏感词。";
SensitiveWordBs wordBs = SensitiveWordBs.newInstance()
.wordDeny(new MyWordDeny())
.wordAllow(new MyWordAllow())
.init();
Assert.assertEquals("[我的自定义敏感词]", wordBs.findAll(text).toString());
```
这里只有 `我的自定义敏感词` 是敏感词,而 `测试` 不是敏感词。
当然,这里是全部使用我们自定义的实现,一般建议使用系统的默认配置+自定义配置。
可以使用下面的方式。
### 同时配置多个
- 多个敏感词
`WordDenys.chains()` 方法,将多个实现合并为同一个 IWordDeny。
- 多个白名单
`WordAllows.chains()` 方法,将多个实现合并为同一个 IWordAllow。
例子:
```java
String text = "这是一个测试。我的自定义敏感词。";
IWordDeny wordDeny = WordDenys.chains(WordDenys.defaults(), new MyWordDeny());
IWordAllow wordAllow = WordAllows.chains(WordAllows.defaults(), new MyWordAllow());
SensitiveWordBs wordBs = SensitiveWordBs.newInstance()
.wordDeny(wordDeny)
.wordAllow(wordAllow)
.init();
Assert.assertEquals("[我的自定义敏感词]", wordBs.findAll(text).toString());
```
这里都是同时使用了系统默认配置,和自定义的配置。
注意:**我们初始化了新的 wordBs,那么用新的 wordBs 去判断。而不是用以前的 `SensitiveWordHelper` 工具方法,工具方法配置是默认的!**
# spring 整合
## 背景
实际使用中,比如可以在页面配置修改,然后实时生效。
数据存储在数据库中,下面是一个伪代码的例子,可以参考 [SpringSensitiveWordConfig.java](https://github.com/houbb/sensitive-word/blob/master/src/test/java/com/github/houbb/sensitive/word/spring/SpringSensitiveWordConfig.java)
要求,版本 v0.0.15 及其以上。
## 自定义数据源
简化伪代码如下,数据的源头为数据库。
MyDdWordAllow 和 MyDdWordDeny 是基于数据库为源头的自定义实现类。
```java
@Configuration
public class SpringSensitiveWordConfig {
@Autowired
private MyDdWordAllow myDdWordAllow;
@Autowired
private MyDdWordDeny myDdWordDeny;
/**
* 初始化引导类
* @return 初始化引导类
* @since 1.0.0
*/
@Bean
public SensitiveWordBs sensitiveWordBs() {
SensitiveWordBs sensitiveWordBs = SensitiveWordBs.newInstance()
.wordAllow(WordAllows.chains(WordAllows.defaults(), myDdWordAllow))
.wordDeny(myDdWordDeny)
// 各种其他配置
.init();
return sensitiveWordBs;
}
}
```
敏感词库的初始化较为耗时,建议程序启动时做一次 init 初始化。
## 动态变更
为了保证敏感词修改可以实时生效且保证接口的尽可能简化,此处没有新增 add/remove 的方法。
而是在调用 `sensitiveWordBs.init()` 的时候,根据 IWordDeny+IWordAllow 重新构建敏感词库。
因为初始化可能耗时较长(秒级别),所有优化为 init 未完成时**不影响旧的词库功能,完成后以新的为准**。
```java
@Component
public class SensitiveWordService {
@Autowired
private SensitiveWordBs sensitiveWordBs;
/**
* 更新词库
*
* 每次数据库的信息发生变化之后,首先调用更新数据库敏感词库的方法。
* 如果需要生效,则调用这个方法。
*
* 说明:重新初始化不影响旧的方法使用。初始化完成后,会以新的为准。
*/
public void refresh() {
// 每次数据库的信息发生变化之后,首先调用更新数据库敏感词库的方法,然后调用这个方法。
sensitiveWordBs.init();
}
}
```
如上,你可以在数据库词库发生变更时,需要词库生效,主动触发一次初始化 `sensitiveWordBs.init();`。
其他使用保持不变,无需重启应用。
# Benchmark
V0.6.0 以后,添加对应的 benchmark 测试。
> [BenchmarkTimesTest](https://github.com/houbb/sensitive-word/blob/master/src/test/java/com/github/houbb/sensitive/word/benchmark/BenchmarkTimesTest.java)
## 环境
测试环境为普通的笔记本:
```
处理器 12th Gen Intel(R) Core(TM) i7-1260P 2.10 GHz
机带 RAM 16.0 GB (15.7 GB 可用)
系统类型 64 位操作系统, 基于 x64 的处理器
```
ps: 不同环境会有差异,但是比例基本稳定。
## 测试效果记录
测试数据:100+ 字符串,循环 10W 次。
| 序号 | 场景 | 耗时 | 备注 |
|:----|:-----------------|:----|:--------------|
| 1 | 只做敏感词,无任何格式转换 | 1470ms,约 7.2W QPS | 追求极致性能,可以这样配置 |
| 2 | 只做敏感词,支持全部格式转换 | 2744ms,约 3.7W QPS | 满足大部分场景 |
# STAR
[](https://starchart.cc/houbb/sensitive-word)
# 后期 road-map
- [x] 移除单个汉字的敏感词,在中国,要把词组当做一次词,降低误判率。
- [ ] 支持单个的敏感词变化?
remove、add、edit?
- [x] 敏感词标签接口支持
- [x] 敏感词处理时标签支持
- [x] wordData 的内存占用对比 + 优化
- [x] 用户指定自定义的词组,同时允许指定词组的组合获取,更加灵活
FormatCombine/CheckCombine/AllowDenyCombine 组合策略,允许用户自定义。
- [ ] word check 策略的优化,统一遍历+转换
- [ ] 添加 ThreadLocal 等性能优化
# 拓展阅读
[01-开源敏感词工具入门使用](https://houbb.github.io/2020/01/07/sensitive-word-00-overview)
[02-如何实现一个敏感词工具?违禁词实现思路梳理](https://houbb.github.io/2020/01/07/sensitive-word-01-intro)
[03-敏感词之 StopWord 停止词优化与特殊符号](https://houbb.github.io/2020/01/07/sensitive-word-02-stopword)
[04-敏感词之字典瘦身](https://houbb.github.io/2020/01/07/sensitive-word-03-slim)
[05-敏感词之 DFA 算法(Trie Tree 算法)详解](https://houbb.github.io/2020/01/07/sensitive-word-04-dfa)
[06-敏感词(脏词) 如何忽略无意义的字符?达到更好的过滤效果](https://houbb.github.io/2020/01/07/sensitive-word-05-ignore-char)
[v0.10.0-脏词分类标签初步支持](https://juejin.cn/post/7308782855941292058?searchId=20231209140414C082B3CCF1E7B2316EF9)
[v0.11.0-敏感词新特性:忽略无意义的字符,词标签字典](https://mp.weixin.qq.com/s/m40ZnR6YF6WgPrArUSZ_0g)
[v0.12.0-敏感词/脏词词标签能力进一步增强](https://mp.weixin.qq.com/s/-wa-if7uAy2jWsZC13C0cQ)
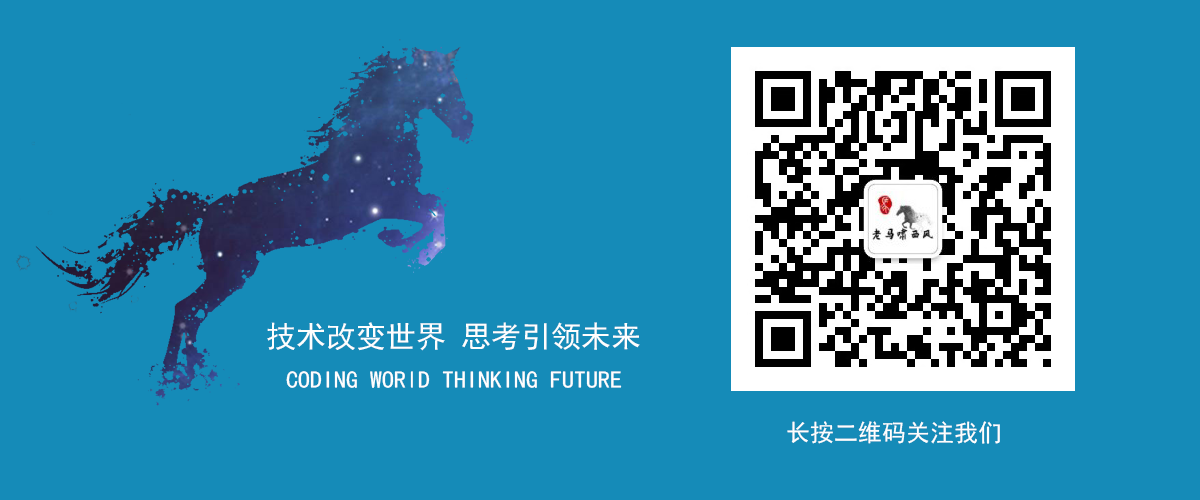
# NLP 开源矩阵
[pinyin 汉字转拼音](https://github.com/houbb/pinyin)
[pinyin2hanzi 拼音转汉字](https://github.com/houbb/pinyin2hanzi)
[segment 高性能中文分词](https://github.com/houbb/segment)
[opencc4j 中文繁简体转换](https://github.com/houbb/opencc4j)
[nlp-hanzi-similar 汉字相似度](https://github.com/houbb/nlp-hanzi-similar)
[word-checker 拼写检测](https://github.com/houbb/word-checker)
[sensitive-word 敏感词](https://github.com/houbb/sensitive-word)
| 0 |
lets-mica/mica | Spring Cloud 微服务开发核心工具集。工具类、验证码、http、redis、ip2region、xss 等,开箱即用。 🔝 🔝 记得右上角点个star 关注更新! | java mica okhttp3 redis spring spring-boot spring-cloud swagger | ## 🌐 mica(云母)
[](https://github.com/lets-mica/mica/actions)

[](https://central.sonatype.com/artifact/net.dreamlu/mica-bom/versions)
[](https://oss.sonatype.org/content/repositories/snapshots/net/dreamlu/)
[](https://www.codacy.com/gh/lets-mica/mica/dashboard?utm_source=github.com&utm_medium=referral&utm_content=lets-mica/mica&utm_campaign=Badge_Grade)
[](https://github.com/lets-mica/mica/blob/master/LICENSE)
`Mica`,Spring Cloud 微服务开发核心包,支持 `web` 和 `webflux`。
简体中文 | [English](README_EN.md)
## 🔖 版本说明
| 最新版本 | mica 版本 | spring boot 版本 | spring cloud 版本 |
|----------|------------------|----------------|-----------------|
| 3.2.4 | mica 3.2.x | 3.2.x | 2023 |
| 3.1.10 | mica 3.1.x | 3.1.x | 2022 |
| 3.0.8 | mica 3.0.x | 3.0.x | 2022 |
| 2.7.18.3 | mica 2.7.x | 2.7.x | 2021 |
| 2.6.8 | mica 2.6.x | 2.6.x | 2021 |
| 2.5.8 | mica 2.5.x | 2.5.x | 2020 |
| 2.4.11 | mica 2.4.x | 2.4.x | 2020 |
| 2.1.1-GA | mica 2.0.x~2.1.x | 2.2.x ~ 2.3.x | Hoxton |
## 🏷️ 版本号说明
`release` 版本号格式为 `x.x.x`, 基本上保持跟 `Spring boot` 一致。
`snapshots` 版本号格式为 `x.x.x-SNAPSHOT`。
`注意`:`snapshots` 版本 `push` 后会自动发布,及时响应修复最新的 `bug` 和需求。
## 📝 使用文档
**使用文档**详见:[https://www.dreamlu.net/mica2x/index.html(mica2.x 使用文档)](https://www.dreamlu.net/mica2x/index.html)
**更新记录**详见:[Mica 更新记录](CHANGELOG.md)
## 🌱 mica 生态
- mica-auto (Spring boot starter 利器): https://gitee.com/596392912/mica-auto
- mica-weixin(jfinal weixin 的 spring boot starter): https://gitee.com/596392912/mica-weixin
- mica-mqtt(基于 t-io 实现的 mqtt组件): https://gitee.com/596392912/mica-mqtt
- Spring cloud 微服务 http2 方案(h2c): https://gitee.com/596392912/spring-cloud-java11
- mica-security(mica权限系统 vue 改造中): https://gitee.com/596392912/mica-security

## 🐛 已知问题
lombok 生成的 method 问题:https://github.com/rzwitserloot/lombok/issues/1861
对于 xX 类属性名,第一个小写,第二个大写的 bean 属性名,Map -> Bean 或 Bean -> Map 存在问题。
不打算做兼容,待 lombok 新版修复。
## 📌 开源协议

**软著编号**:`2020SR0411603`
### 📄 协议解释
LGPL([GNU Lesser General Public License](http://www.gnu.org/licenses/lgpl.html))
LGPL 是 GPL 的一个为主要为类库使用设计的开源协议。和 GPL 要求任何使用/修改/衍生之 GPL 类库的的软件必须采用 GPL 协议不同。LGPL 允许商业软件通过类库引用(link)方式使用 LGPL 类库而不需要开源商业软件的代码。这使得采用 LGPL 协议的开源代码可以被商业软件作为类库引用并发布和销售。
但是如果修改 LGPL 协议的代码或者衍生,则所有修改的代码,涉及修改部分的额外代码和衍生的代码都必须采用 LGPL 协议。因此 LGPL 协议的开源代码很适合作为第三方类库被商业软件引用,但不适合希望以 LGPL 协议代码为基础,通过修改和衍生的方式做二次开发的商业软件采用。
### ✅ 用户权益
允许以引入不改源码的形式免费用于学习、毕设、公司项目、私活等。
特殊情况修改代码,但仍然想闭源需经过作者同意。
对未经过授权和不遵循 LGPL 协议二次开源或者商业化我们将追究到底。
参考请注明:参考自 mica:https://github.com/lets-mica/mica ,另请遵循 LGPL 协议。
`注意`:若禁止条款被发现有权追讨 **19999** 的授权费。
### 🗃️ 授权用户(最佳实践)
- `pigx` 宇宙最强微服务(架构师必备):https://pig4cloud.com
- `bladex` 完整的线上解决方案(企业生产必备):https://bladex.vip
## 🔍️ 相关链接
- mica 源码 Github:[https://github.com/lets-mica](https://github.com/lets-mica)
- mica 源码 Gitee(码云):[https://gitee.com/596392912/mica](https://gitee.com/596392912/mica)
- mica 性能压测:[https://github.com/lets-mica/mica-jmh](https://github.com/lets-mica/mica-jmh)
- 文档地址(官网):[http://wiki.dreamlu.net](http://wiki.dreamlu.net/guide/getting-started.html)
- 文档地址(语雀-可关注订阅):[https://www.yuque.com/dreamlu](https://www.yuque.com/dreamlu)
## 🍻 开源推荐
- `Avue` 一款基于 vue 可配置化的神奇框架:[https://gitee.com/smallweigit/avue](https://gitee.com/smallweigit/avue)
- `pig` 宇宙最强微服务(架构师必备):[https://gitee.com/log4j/pig](https://gitee.com/log4j/pig)
- `SpringBlade` 完整的线上解决方案(企业开发必备):[https://gitee.com/smallc/SpringBlade](https://gitee.com/smallc/SpringBlade)
- `IJPay` 支付 SDK 让支付触手可及:[https://gitee.com/javen205/IJPay](https://gitee.com/javen205/IJPay)
- `JustAuth` 史上最全的整合第三方登录的开源库: [https://github.com/zhangyd-c/JustAuth](https://github.com/zhangyd-c/JustAuth)
- `spring-boot-demo` 深度学习并实战 spring boot 的项目: [https://github.com/xkcoding/spring-boot-demo](https://github.com/xkcoding/spring-boot-demo)
## 🍱 赞助商
[](https://gitee.com/dromara/MaxKey/?from=mica-mqtt)
## 💚 鸣谢
感谢 JetBrains 提供的免费开源 License:
[](https://www.jetbrains.com/?from=mica)
感谢 `如梦技术 VIP` **小伙伴们**的鼎力支持,更多 **VIP** 信息详见:https://www.dreamlu.net/vip/index.html
## 🍱 赞助计划
mica 始于一份热爱,也得到不少朋友的认可,为了更好的发展,特推出赞助计划。**知识付费**,让你我走的更远!!!
| 类型 | ¥ | 权益(永久) |
| ---------- | ---- |-------------------------------|
| 青铜赞助人 | 199 | mica 最佳使用范例 mica-less 权限系统源码。 |
| 黄金赞助人 | 599 | 原如梦VIP,享受如梦技术原微服务相关资源。 |
**注意:** 加微信 **DreamLuTech** 详聊。
## 📱 微信公众号

**JAVA架构日记**,精彩内容每日推荐!
| 0 |
mxdldev/spring-cloud-flycloud | 🔥🔥🔥FlyClould 微服务实战项目框架,在该框架中,包括了用 Spring Cloud 构建微服务的一系列基本组件和框架,对于后台服务框架的搭建有很大的参考价值,大家可以参考甚至稍加修改可以直接应用于自己的实际的项目开发中,该项目没有采用Maven进行项目构建,Maven通过xml进行依赖管理,导致整个配置文件太过臃肿,另外灵活性也不是很强,所以我采用Gradle进行项目构建和依赖管理,在FlyTour项目中我们见证了Gradle的强大,通过简单的一些配置就可以轻松的实现组件化的功能。该项目共有11个Module工程。其中10个位微服务工程,这10个微服务工程构成了一个完整的微服务系统,微服务系统包含了8个基础服务,提供了一整套微服务治理功能,他们分别是配置中心module_config、注册中心module_eureka、认证授权中心module_uaa、Turbine聚合监控服务module_monitor、链路追踪服务module_zipken、聚合监控服务module_admin、路由网关服务module_gateway、日志服务module_log。另外还包含了两个资源服务:用户服务module_user和blog服务module_blog,另外还有一个common的Module,为资源服务提供一些一本的工具类 | spring spring-boot spring-cloud spring-data-jpa spring-mvc spring-security springboot springframework springmvc | # FlyCloud
FlyClould 微服务实战项目框架,在该框架中,包括了用 Spring Cloud 构建微服务的一系列基本组件和框架,对于后台服务框架的搭建有很大的参考价值,大家可以参考甚至稍加修改可以直接应用于自己的实际的项目开发中
csdn地址:[https://blog.csdn.net/geduo_83/article/details/87866018](https://blog.csdn.net/geduo_83/article/details/87866018)
欢迎点赞加星,评论打call、加群:810970432
在该框架中包括了用 Spring Cloud 构建微服务的一系列基本组件和框架,对于后台服务框架的搭建有很大的参考价值,大家可以参考甚至稍加修改可以直接应用于自己的实际的项目开发中。
### 更新日志:
### [FlyCloud 3.0.0](https://github.com/mxdldev/spring-cloud-flycloud/releases) 2020-03-23
为了满足广大开发者的便于测试、便于部署需求,对FlyCloud进行了单一结构体改造,并将其部署在了外网上,接口文档如下:[http://106.12.61.238:8080/swagger-ui.html](http://106.12.61.238:8080/swagger-ui.html)
* spring boot + security + oauth2 单一结构体改造
* 将所有的访问接口开放在外网上
### [FlyCloud 2.2.0](https://github.com/mxdldev/spring-cloud-flycloud/releases) 2020-03-09
* springboot 升级为2.0.5
### [FlyCloud 2.1.0](https://github.com/mxdldev/spring-cloud-flycloud/releases) 2019-07-01
* 相关bug修改
* 代码优化
### [FlyCloud 2.0.0](https://github.com/mxdldev/spring-cloud-flycloud/releases) 2019-06-08
* 业务组件module_news添加
### [FlyCloud 1.0.0](https://github.com/mxdldev/spring-cloud-flycloud/releases) 2019-02-28
初始版本:
* 1.配置组件:注册中心、配置中心、授权中心
* 2.监控组件:聚合监控、熔断监控、路由监控
* 3.其他组件:网关服务、日志服务、公共服务
* 4.业务组件:用户组件,博客组件
### 开发环境:
在该框架中,包括了用 Spring Cloud 构建微服务的一系列基本组件和框架,运行所需要软件如下:
* 开发IDE:IDEA
https://www.jetbrains.com/idea/download/#section=windows
* 数据库:mysql5.7
https://dev.mysql.com/downloads/mysql/
* 组件通信rabbitmq
http://www.rabbitmq.com/download.html
* Erlang环境
http://www.erlang.org/downloads Erlang环境
http://www.cnerlang.com/resource/182.html
该项目没有采用Maven进行项目构建,Maven通过xml进行依赖管理,导致整个配置文件太过臃肿,另外灵活性也不是很强,所以我采用Gradle进行项目构建和依赖管理,在FlyTour项目中我们见证了Gradle的强大,通过简单的一些配置就可以轻松的实现组件化的功能。该项目共有11个Moudle工程。其中10个位微服务工程,这10个微服务工程构成了一个完整的微服务系统,微服务系统包含了8个基础服务,提供了一整套微服务治理功能,他们分别是配置中心module_config、注册中心module_eureka、认证授权中心module_uaa、Turbine聚合监控服务module_monitor、链路追踪服务module_zipken、聚合监控服务module_admin、路由网关服务module_gateway、日志服务module_log。另外还包含了两个资源服务:用户服务module_user和blog服务module_blog,另外还有一个common的Moudle,为资源服务提供一些一本的工具类。
### 工程架构图:
<br>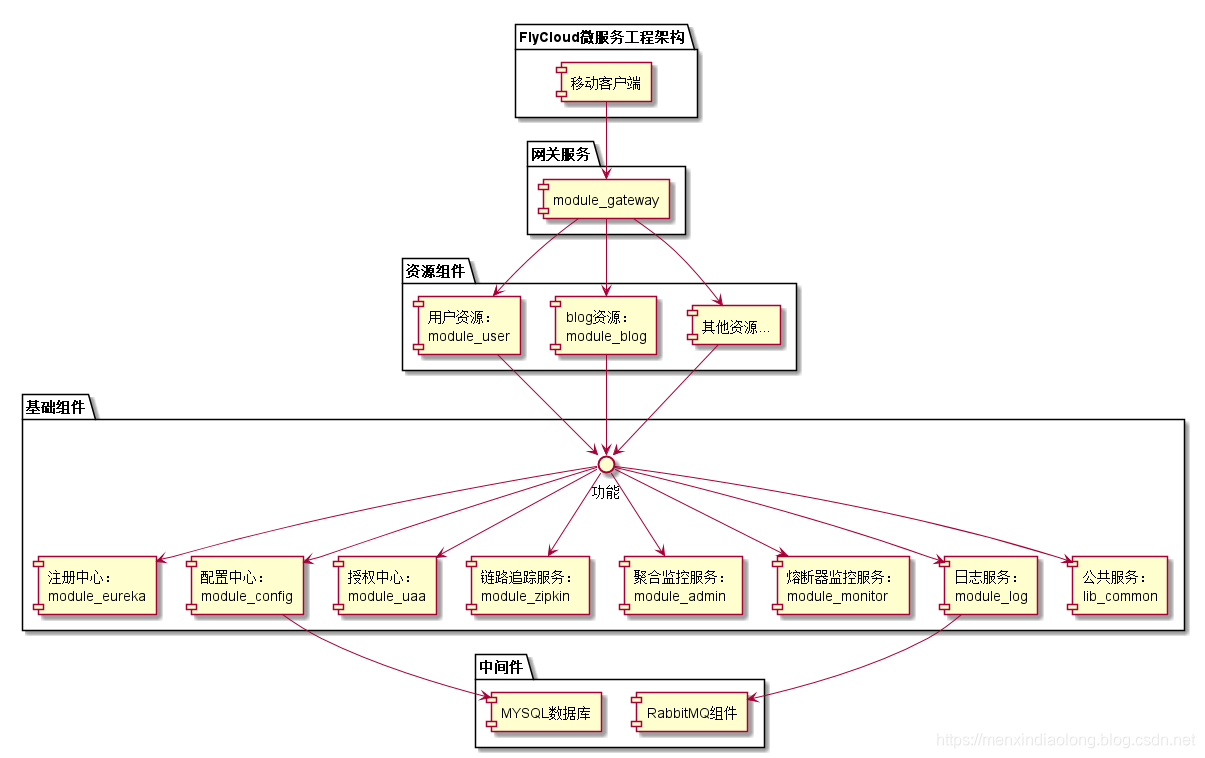
<br>下面对11个Moudle工程分别进行介绍:
* 1.注册中心:module_eureka
在这个系统中,所有的服务都向注册中心module_eureka进行服务注册。能方便的查看每个服务的服务状况、服务是否可用,以及每个服务都有哪些服务实例
工作流程:
<br>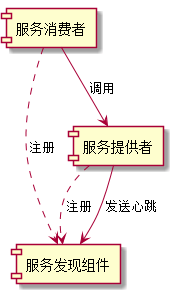
* 2.配置中心:module_config
配置中心所有服务的配置文件由 config-server 管理,特别说明为了简单起见本框架中配置数据都放在本地并没有从git仓库远程获取
架构图:
<br>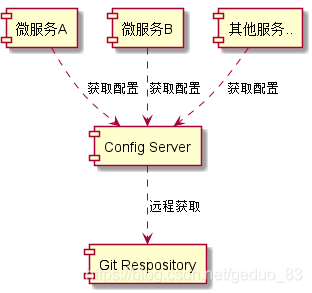
* 3.网关服务:module_gateway
网关服务使用的是 Zuul 组件, Zuul 组件可以实现智能路由、负载均衡的功能 gateway-service 作为 个边界服务,对外统一暴露 API 接口,其他的服务 API 接口只提供给内部服务调用,不提供给外界直接调用,这就很方便实现统鉴权、安全验证的功能
通过路由网关实现负载均衡:
<br>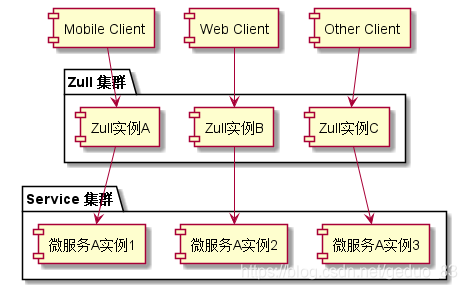
* 4.链路追踪服务:module_zipkin
它可以查看每个请求在微服务系统中的链路关系
* 5.聚合监控服务:module_admin
提供了非常强大的服务监控功能,可以查看每个向注册中心注册服务的健康状态, 日志、注册时间等
* 6.Turbine聚合监控服务:module_monitor
它聚合了 module_user和module_blog的Hystrix Dashboard ,可以查看这两个服务熔断器的监控状况
* 7.认证授权服务:module_uaa
Spring Cloud 0Auth2 由这个服务统一授权并返回Token。其他的应用服务例如module_user和module_blog作为资源服务 API 接口资源受保护的,需要验证Token并鉴后才能访问,我采用的0Auth2+JWT安全认证,需要生成私钥用于加密,公钥用于解密
生成私钥命令:
```
keytool -genkeypair -alias fly-jwt -validity 36500 -keyalg RSA -dname "CN=jwt,OU=jwt,O=jwt,L=haidian,S=beijing,C=CH" -keypass fly123 -keystore fly-jwt.jks -storepass fly123
```
生成公钥命令:
```
keytool -list -rfc --keystore fly-jwt.jks | openssl x509 -inform pem -pubkey
```
JWT认证流程:
<br>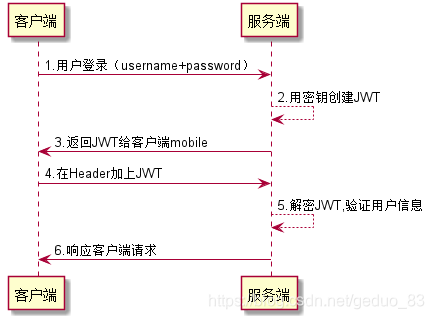
* 8.用户服务:module_user
作为资源服务,对外暴露用户的API接口资源
* 9.blog服务:module_blog
作为资源服务,对外暴露blog的API接口资源
* 10.日志服务:module_log
作为日志服务, module_user和module_blog服务通过RabbitMQ向module_log发送业务操作日志的消息,最后统一保存在数据库,由它统一持久化操作日志
日志服务架构图:
<br>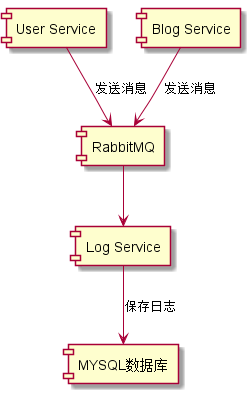
### 功能演示:
依次启动 module_eureka, module_config,module_zipkin及其他的微服务,等整个微服务系统完全启动之后,在览器上访问 即:http://localhost:8761,即就是Eureka 可以查看服务注册的情况
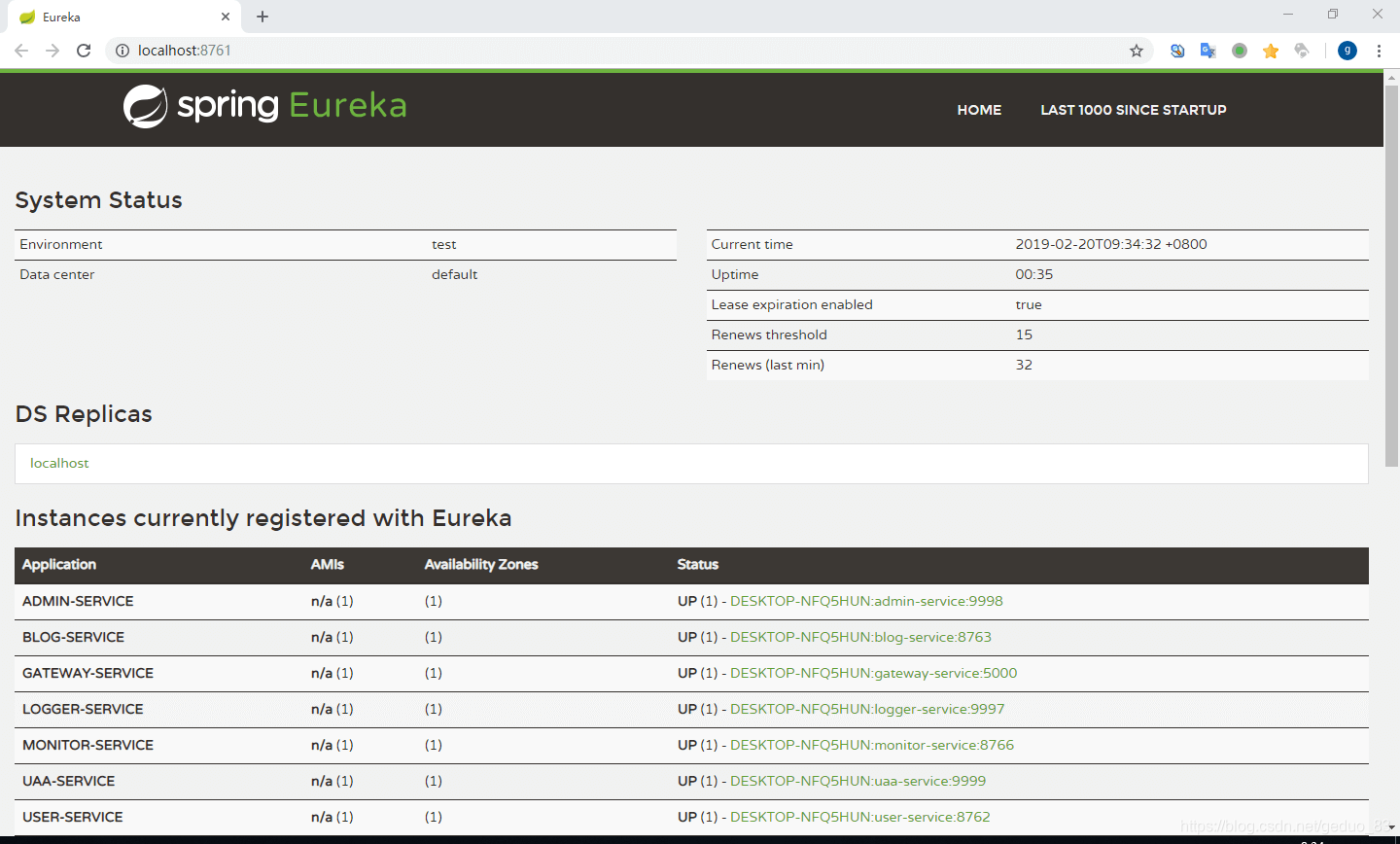
API 接口文档采用 Swagger2 框架生成在线文档, module_user 工程和 module_blog工程集成了Swagger2 ,集成Swagger2 只需要引入依赖,并做相关的配置,然后在具体的 Controller上写注解,就可以实现 Swagger2的在线文档功能在浏览器输入http://localhost:8762/swagger-ui.html 查看user服务的api文档
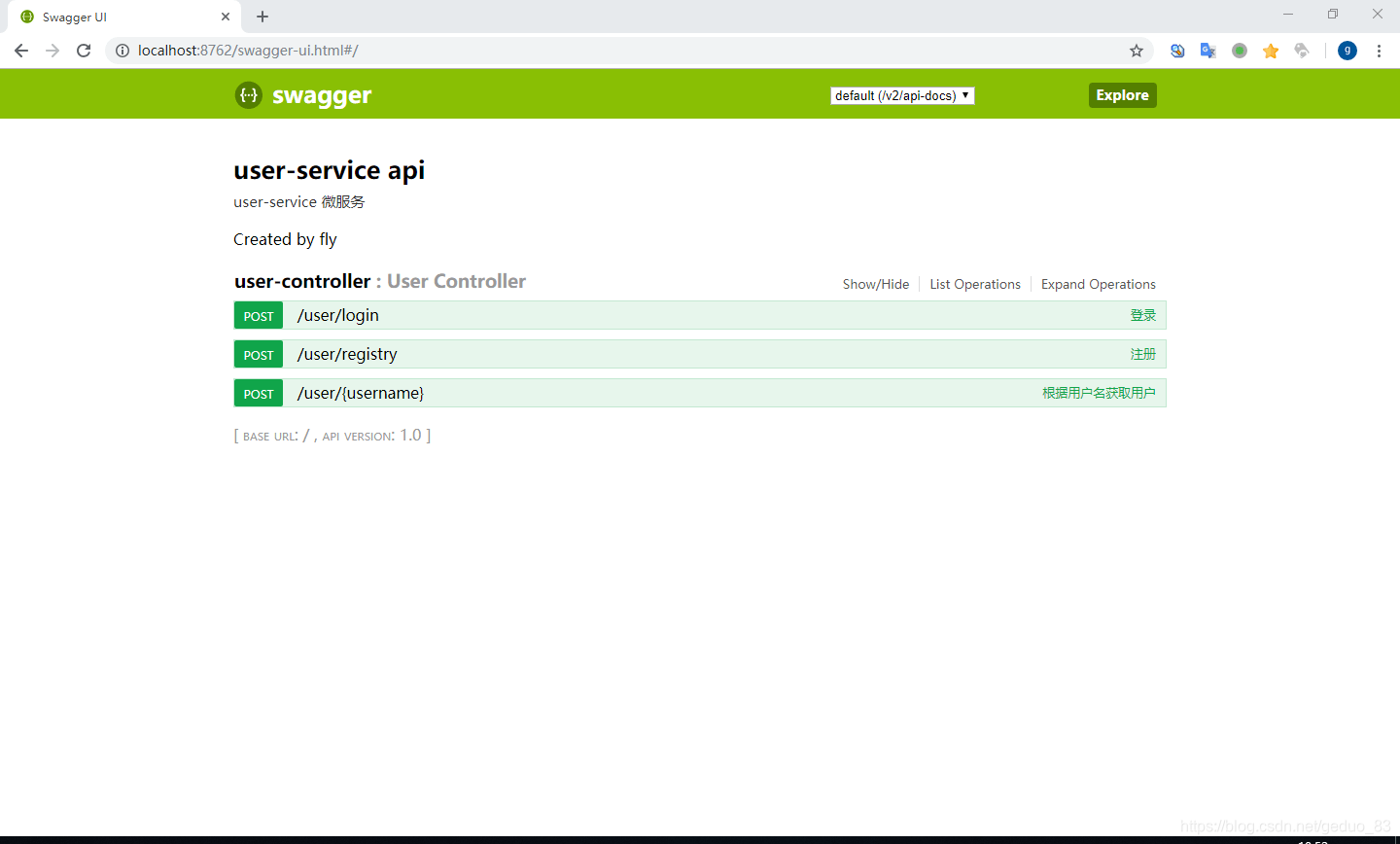
在浏览器输入http://localhost:8763/swagger-ui.html 查看blog服务的api文档
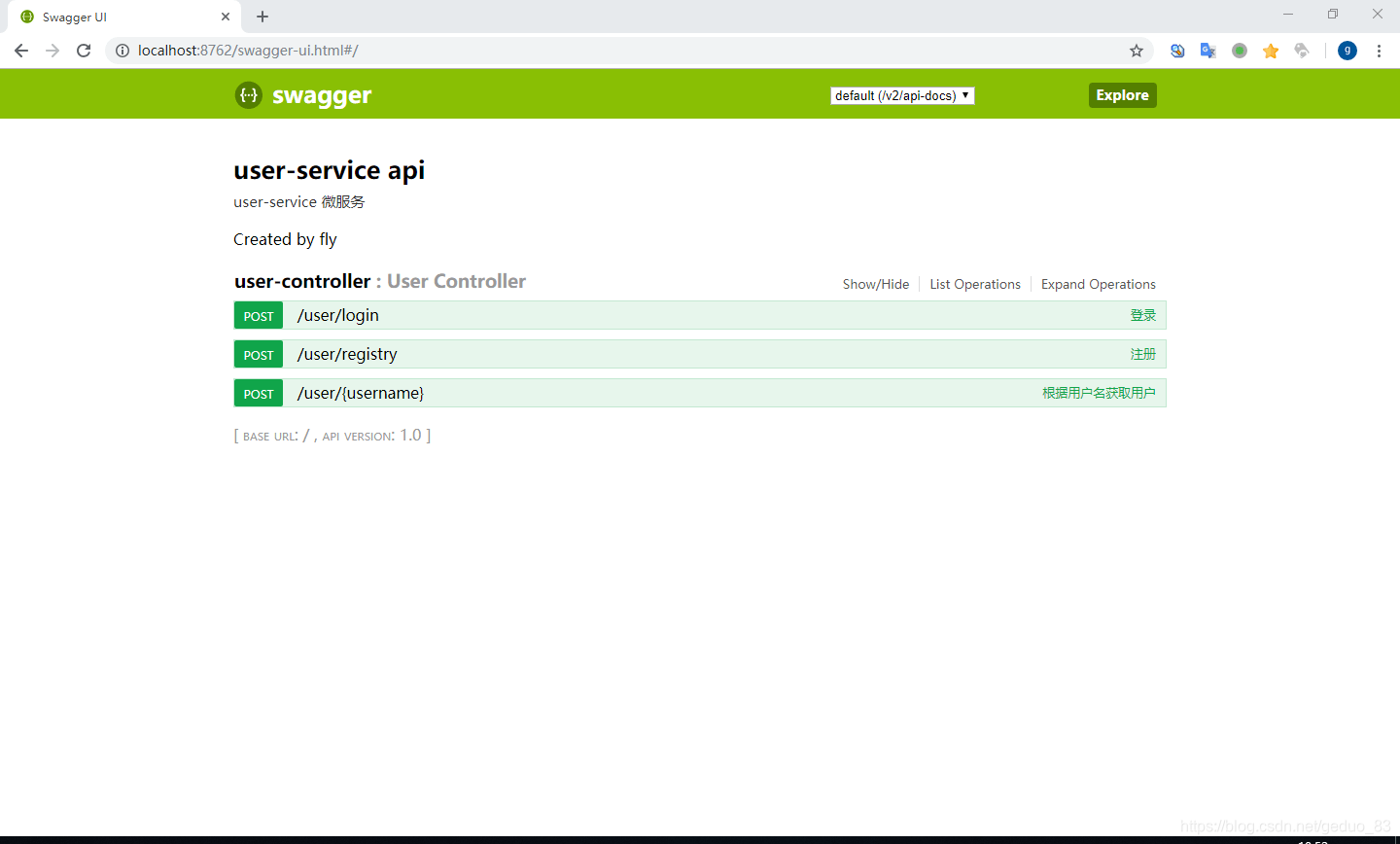
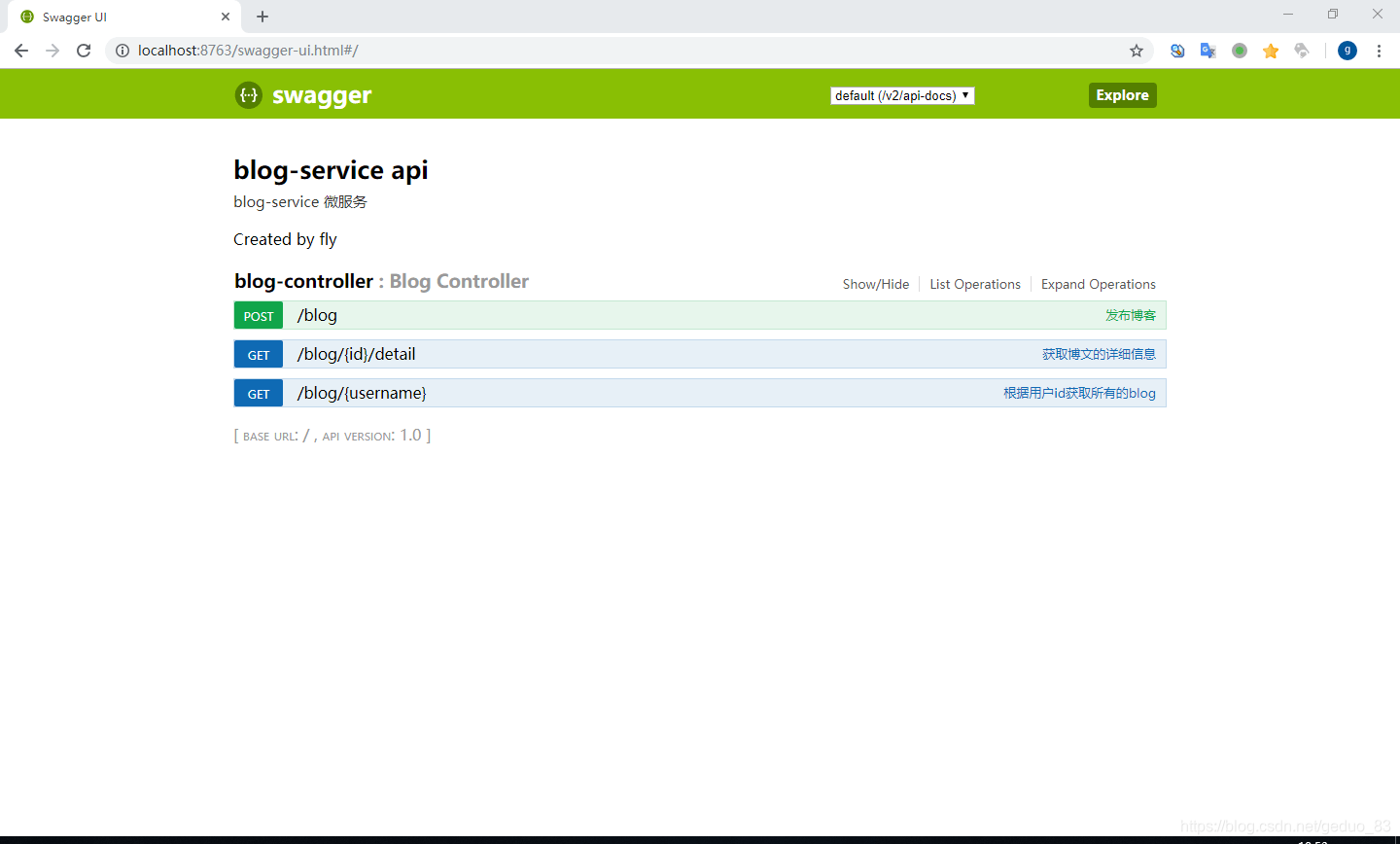
在浏览器上访问 http://localhost :9998 展示了 admin-service 登录界面,admin-service 作为 个综合监控的服务,需要对访问者进行身份认证才能访问它的主页,登录用户名为 dmin 密码为 123456
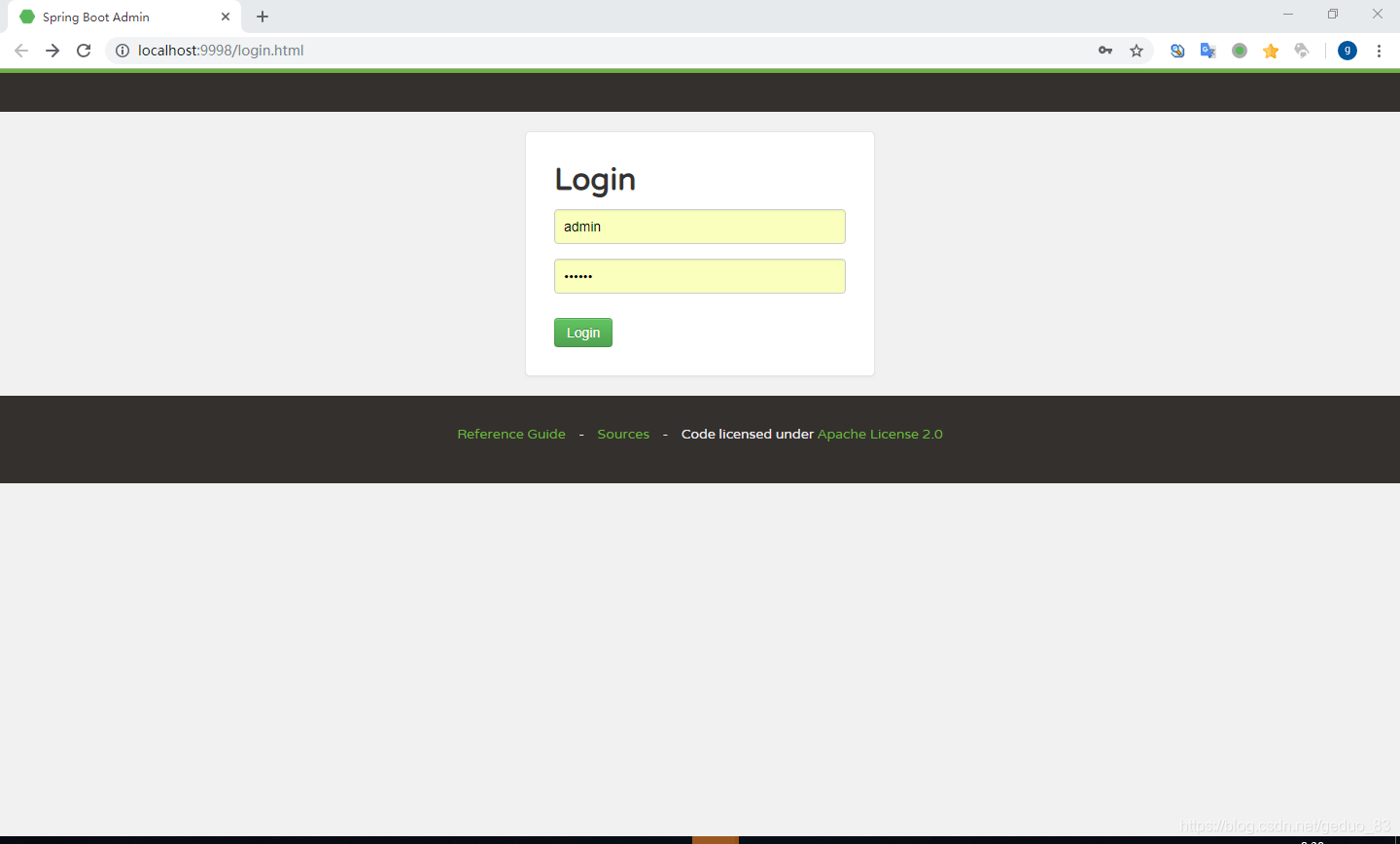
这是登录成功后进入的首页面:
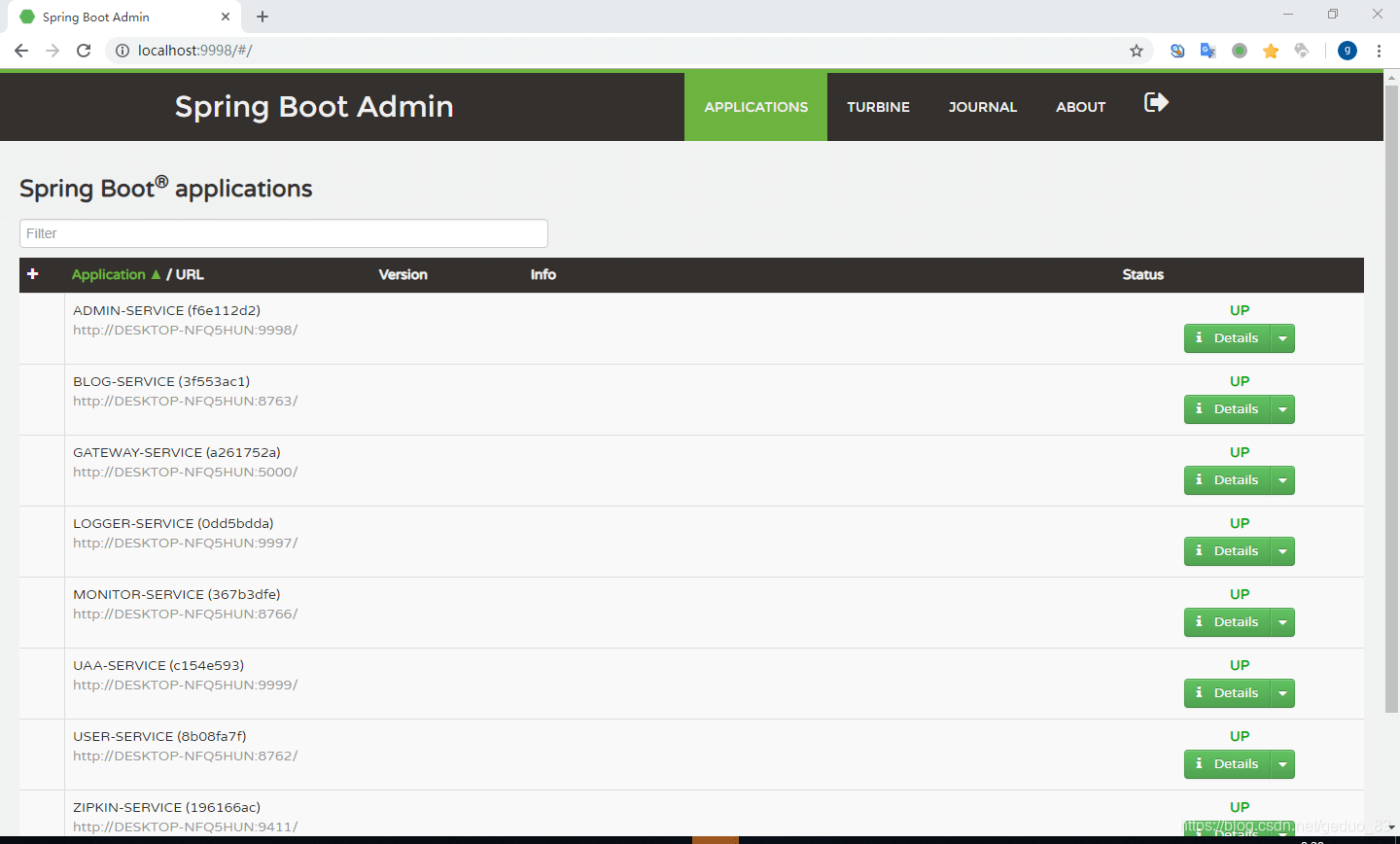
点击TURBINE,这是user服务和blog服务的熔断器监控页面
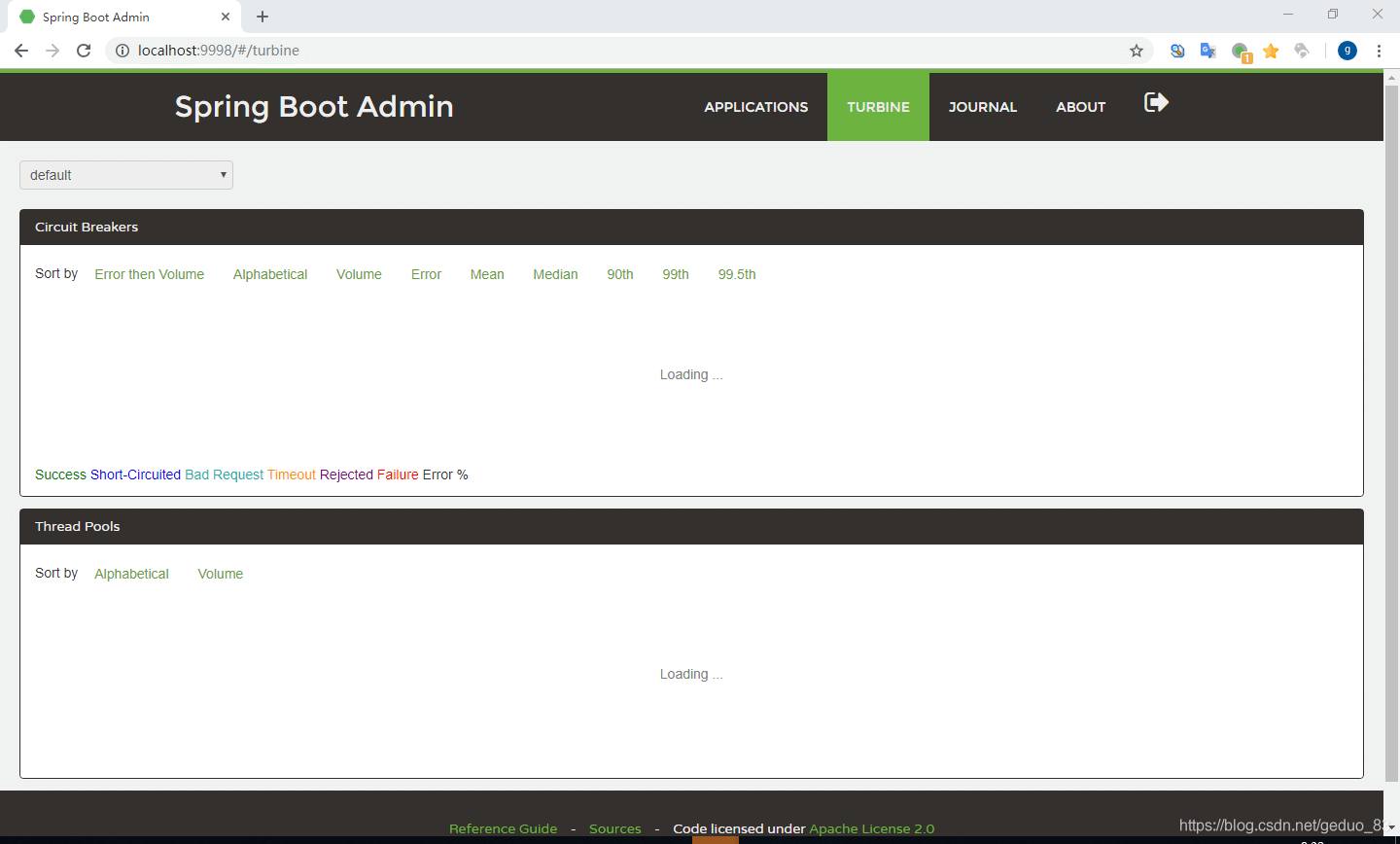
在浏览器上访问 http://localhost :9411查看服务之间相互调用的链路追踪
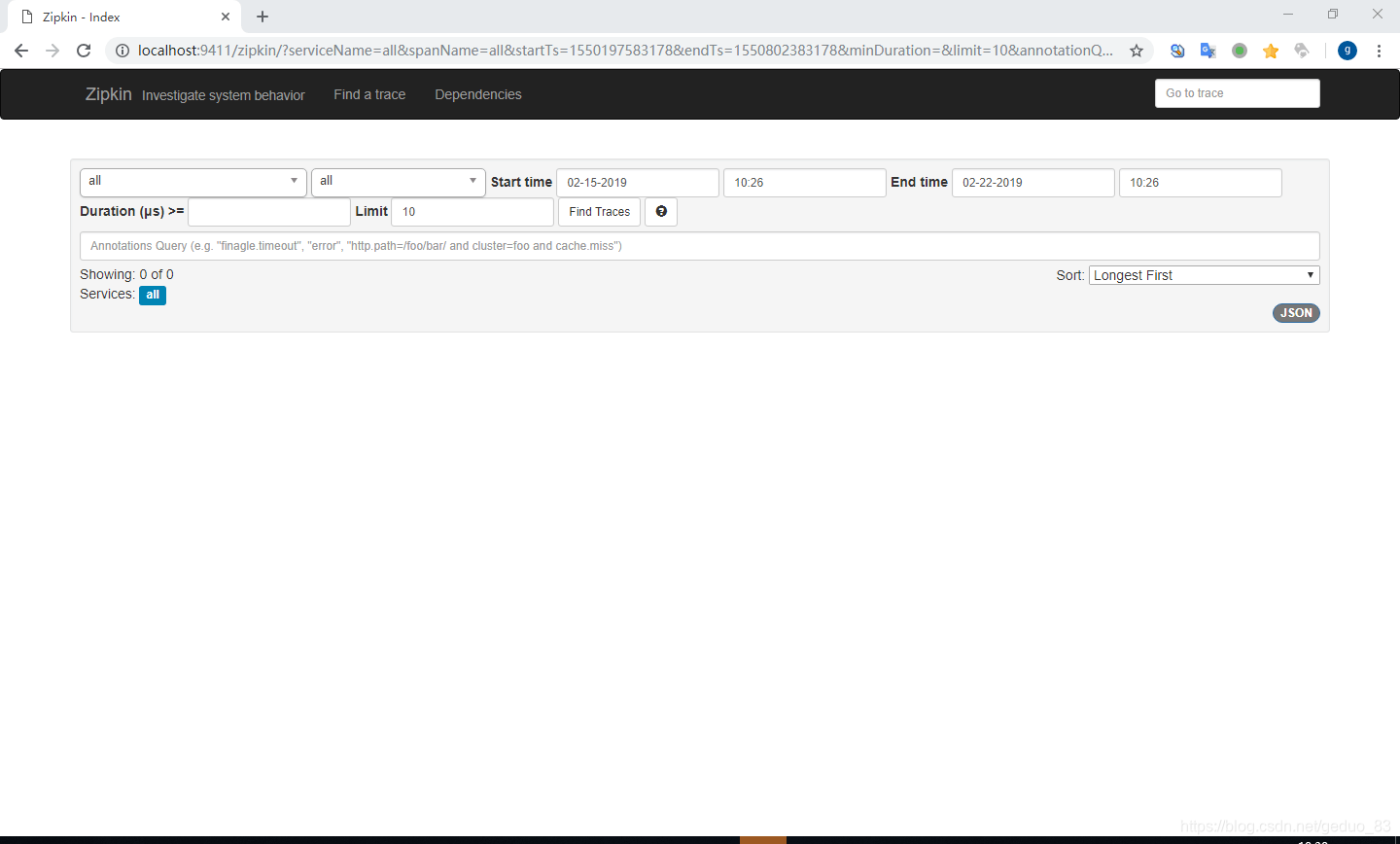
好了,截止到现在整个项目就全部介绍完了,本项目可以直接拿来应用,进行项目开发,本框架代码量较大,所以我就不贴源代码了
### 问题反馈
在使用中有任何问题,请在下方留言,或加入Android、Java开发技术交流群
QQ群:810970432
email:geduo_83@163.com
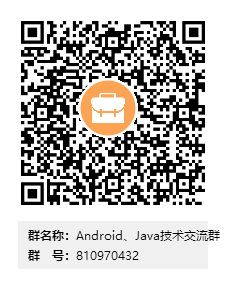
### 关于作者
```
var mxdl = {
name : "门心叼龙",
blog : "https://menxindiaolong.blog.csdn.net"
}
```
### License
```
Copyright (C) menxindiaolong, FlyCloud Framework Open Source Project
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
```
| 0 |
camunda/camunda-bpm-examples | A collection of usage examples for Camunda Platform intended to get you started quickly | null | Camunda Platform examples
====================
> Looking for the "invoice" example contained in the distribution? You can find it here: https://github.com/camunda/camunda-bpm-platform/tree/master/examples/invoice
Camunda Platform examples is a collection of focused usage examples for the [Camunda Platform](https://github.com/camunda/camunda-bpm-platform), intended to get you started quickly. The sources on the master branch work with the current Camunda release. Follow the links below to browse the examples for the Camunda version you use:
| Camunda Version | Link | Checkout command |
|-----------------|-----------------------------------------------------------------------|-----------------------|
| Latest | [Master branch](https://github.com/camunda/camunda-bpm-examples) | `git checkout master` |
| 7.21 | [7.21 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.21) | `git checkout 7.21` |
| 7.20 | [7.20 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.20) | `git checkout 7.20` |
| 7.19 | [7.19 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.19) | `git checkout 7.19` |
| 7.18 | [7.18 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.18) | `git checkout 7.18` |
| 7.17 | [7.17 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.17) | `git checkout 7.17` |
| 7.16 | [7.16 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.16) | `git checkout 7.16` |
| 7.15 | [7.15 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.15) | `git checkout 7.15` |
| 7.14 | [7.14 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.14) | `git checkout 7.14` |
| 7.13 | [7.13 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.13) | `git checkout 7.13` |
| 7.12 | [7.12 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.12) | `git checkout 7.12` |
| 7.11 | [7.11 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.11) | `git checkout 7.11` |
| 7.10 | [7.10 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.10) | `git checkout 7.10` |
| 7.9 | [7.9 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.9) | `git checkout 7.9` |
| 7.8 | [7.8 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.8) | `git checkout 7.8` |
| 7.7 | [7.7 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.7) | `git checkout 7.7` |
| 7.6 | [7.6 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.6) | `git checkout 7.6` |
| 7.5 | [7.5 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.5) | `git checkout 7.5` |
| 7.4 | [7.4 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.4) | `git checkout 7.4` |
| 7.3 | [7.3 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.3) | `git checkout 7.3` |
| 7.2 | [7.2 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.2) | `git checkout 7.2` |
| 7.1 | [7.1 tag](https://github.com/camunda/camunda-bpm-examples/tree/7.1) | `git checkout 7.1` |
If you clone this repository, use the checkout commands to access the sources for the desired version.
## Overview
* [Getting Started with Camunda Platform](#getting-started-with-camunda-platform)
* [BPMN 2.0 & Process Implementation](#bpmn-20--process-implementation-examples)
* [Deployment & Project Setup](#deployment--project-setup-examples)
* [Process Engine Plugin](#process-engine-plugin-examples)
* [Bpmn 2.0 Model API](#bpmn-20-model-api-examples)
* [Cmmn 1.1 Model API Examples](#cmmn-11-model-api-examples)
* [Cockpit](#cockpit-examples)
* [Tasklist](#tasklist-examples)
* [Multi-Tenancy](#multi-tenancy-examples)
* [Spin](#spin-examples)
* [DMN](#dmn-examples)
* [Process Instance Migration](#process-instance-migration-examples)
* [SDK-JS Examples](#sdk-js-examples)
* [Authentication](#authentication)
* [Spring Boot Starter examples](#spring-boot-starter-examples)
* [Quarkus Extension Examples](#quarkus-extension-examples)
* [External Task Client](#external-task-client)
* [External Task Client Spring](#external-task-client-spring)
* [External Task Client Spring Boot](#external-task-client-spring-boot)
* [Testing](#testing)
### Getting Started with Camunda Platform
| Name | Container |
|------------------------------------------------------------------------------------------------|--------------------|
| [Simple Process Applications](https://docs.camunda.org/get-started/bpmn20/) | All |
| [Camunda Platform and the Spring Framework](https://docs.camunda.org/get-started/spring/) [^1] | Tomcat |
| [Process Applications with JavaEE 6](https://docs.camunda.org/get-started/javaee6/) [^1] | JavaEE Containers |
### BPMN 2.0 & Process Implementation Examples
| Name | Container | Keywords |
|------------------------------------------------------------------------------------------------------------------|-------------------|---------------------------|
| [Service Task REST HTTP](/servicetask/rest-service) | Unit Test | Rest Scripting, classless |
| [Service Task SOAP HTTP](/servicetask/soap-service) [^1] | Unit Test | SOAP Scripting, classless |
| [Service Task SOAP CXF HTTP](/servicetask/soap-cxf-service) [^1] | Unit Test | SOAP, CXF, Spring, Spin |
| [Service Invocation Synchronous](/servicetask/service-invocation-synchronous) | Unit Test | Java Delegate, Sync |
| [Service Invocation Asynchronous](/servicetask/service-invocation-asynchronous) | Unit Test | Signal, Async |
| [User Task Assignment Email](/usertask/task-assignment-email) [^1][^2] | All | Email, Usertask |
| [User Task Form Embedded](/usertask/task-form-embedded) [^2] | All | Html, Form, Usertask |
| [User Task Form Embedded - Serialized Java Object](/usertask/task-form-embedded-serialized-java-object) [^1][^2] | All | Html, Form, Usertask |
| [User Task Form Embedded - JSON](/usertask/task-form-embedded-json-variables) [^2] | All | Html, Form, Usertask |
| [User Task Form Embedded - Bpmn Elements](/usertask/task-form-embedded-bpmn-events) [^2] | All | Html, Form, Usertask |
| [User Task Form Embedded - React](/usertask/task-form-embedded-react) [^2] | All | Html, Form, Usertask |
| [User Task Form - Camunda Forms](/usertask/task-camunda-forms) [^2] | All | Html, Form, Usertask |
| [User Task Form Generated](/usertask/task-form-generated) [^1][^2] | All | Html, Form, Usertask |
| [User Task Form JSF](/usertask/task-form-external-jsf) [^1][^2] | JavaEE Containers | JSF, Form, Usertask |
| [Script Task XSLT](/scripttask/xslt-scripttask) | Unit Test | XSLT Scripttask |
| [Script Task XQuery](/scripttask/xquery-scripttask) [^1] | Unit Test | XQuery Scripttask |
| [Start Event - Message](/startevent/message-start) | Unit Test | Message Start Event |
| [Start Process - SOAP CXF](/startevent/soap-cxf-server-start) [^1] | War | SOAP, CXF, Spring |
### Deployment & Project Setup Examples
| Name | Container | Keywords |
|-------------------------------------------------------------------------------------------------|-----------------------|---------------------------|
| [Process Application - Servlet](deployment/servlet-pa) | All | War, Servlet |
| [Process Application - EJB](deployment/ejb-pa) | JavaEE Containers | Ejb, War |
| [Process Application - Jakarta EJB](deployment/ejb-pa-jakarta) | Jakarta EE Containers | Ejb, War |
| [Process Application - Spring 5 Servlet - WildFly](deployment/spring-servlet-pa-wildfly) | WildFly | Spring, Servlet, War |
| [Process Application - Spring 5 Servlet - Embedded Tomcat](deployment/spring-servlet-pa-tomcat) | Tomcat | Spring, Servlet, War |
| [Embedded Spring 5 with embedded REST](deployment/embedded-spring-rest) | vanilla Apache Tomcat | Spring, Rest, Embedded |
| [Plain Spring 5 Web application - WildFly](deployment/spring-wildfly-non-pa) | WildFly | Spring, Jndi, War |
| [Process Application - Spring Boot](deployment/spring-boot) [^1] | Spring Boot | Spring |
### Process Engine Plugin Examples
| Name | Container | Keywords |
|-------------------------------------------------------------------------------------------------|----------------------|---------------------------------------------|
| [BPMN Parse Listener](process-engine-plugin/bpmn-parse-listener) | Unit Test | Process Engine Plugin, Bpmn Parse Listener |
| [BPMN Parse Listener on User Task](process-engine-plugin/bpmn-parse-listener-on-user-task) [^1] | Unit Test | Process Engine Plugin, Bpmn Parse Listener |
| [Command Interceptor - Blocking](process-engine-plugin/command-interceptor-blocking) | Unit Test | Maintenance, Interceptor, Configuration |
| [Custom History Level](process-engine-plugin/custom-history-level) | Unit Test | Process Engine Plugin, Custom History Level |
| [Failed Job Retry Profile](process-engine-plugin/failed-job-retry-profile) | Unit Test | Process Engine Plugin, Failed Job Retry |
### Bpmn 2.0 Model API Examples
| Name | Container | Keywords |
|----------------------------------------------------------------------|----------------------|---------------------------|
| [Generate JSF forms](/bpmn-model-api/generate-jsf-form) [^1] | JavaEE Containers | JSF, Usertask |
| [Generate BPMN process](/bpmn-model-api/generate-process-fluent-api) | Unit Test | Fluent API |
| [Parse BPMN model](/bpmn-model-api/parse-bpmn) | Unit Test | BPMN |
### Cmmn 1.1 Model API Examples
| Name | Container | Keywords |
|--------------------------------------------------------------------------------------------------|----------------------|---------------------------|
| [Strongly-typed Access to Custom Extension Elements](/cmmn-model-api/typed-custom-elements) [^1] | Unit Test | CMMN, TransformListener |
### Cockpit Examples
| Name | Keywords |
|----------------------------------------------------------------------------------------------------------------|-------------------------------------------|
| [Fullstack (ReactJS 16.x & Java) "Count Processes" Cockpit Plugin](/cockpit/cockpit-fullstack-count-processes) | Plugin, Custom Script, Fullstack, ReactJS |
| [Angular "Open Usertasks" Cockpit Tab](/cockpit/cockpit-angular-open-usertasks) [^1] | Plugin, Custom Script, Angular |
| [AngularJS 1.x "Search Processes" Cockpit Plugin](/cockpit/cockpit-angularjs-search-processes) [^1] | Plugin, Custom Script, AngularJS |
| [ReactJS "Involved Users" Cockpit Plugin](/cockpit/cockpit-react-involved-users) | Plugin, Custom Script, ReactJS |
| ["Cats" Cockpit Plugin](/cockpit/cockpit-cats) [^1] | Plugin, Custom Script |
| ["Diagram interactions" Cockpit Plugin](/cockpit/cockpit-diagram-interactions) | Plugin, Custom Script |
| ["Open Incidents" Cockpit Plugin](/cockpit/cockpit-open-incidents) [^1] | Plugin, Custom Script |
| ["Request Interceptor" Cockpit Script](/cockpit/cockpit-request-interceptor) [^1] | Plugin, Custom Script |
| [bpmn-js Cockpit module](/cockpit/cockpit-bpmn-js-module) [^1] | Plugin, Custom Script, bpmn-js |
| [bpmn-js Cockpit module - bundled](/cockpit/cockpit-bpmn-js-module-bundled) | Plugin, Custom Script, bpmn-js, rollup |
### Tasklist Examples
| Name | Keywords |
|-------------------------------------------------------------------------------------------------------------------------------------------------------------------------|---------------------------|
| [Create Standalone Task - client side](https://github.com/camunda/camunda-bpm-platform/tree/master/webapps/frontend/ui/tasklist/plugins/standaloneTask/app) | Plugin |
| [Create Standalone Task - server side](https://github.com/camunda/camunda-bpm-platform/blob/master/webapps/assembly/src/main/java/org/camunda/bpm/tasklist/impl/plugin) | Plugin |
| [Javascript Only Plugin](/tasklist/cats-plugin) | Plugin, Custom Script |
| [JQuery 3.4 Behavior Patch](/tasklist/jquery-34-behavior) [^1] | Plugin, Custom Script |
### SDK-JS Examples
| Name | Environment | Keywords |
|------------------------------------------------|-------------|-----------------------------|
| [SDK JS forms](/sdk-js/browser-forms) [^1][^2] | Browser | HTML, task, form, SDK |
### Multi-Tenancy Examples
| Name | Container | Keywords |
|----------------------------------------------------------------------------------------------------------------------------|-----------|---------------|
| [Multi-Tenancy with Database Schema Isolation](multi-tenancy/schema-isolation) | Wildfly | Multi-Tenancy |
| [Multi-Tenancy with Tenant Identifiers for Embedded Process Engine](multi-tenancy/tenant-identifier-embedded) | Unit Test | Multi-Tenancy |
| [Multi-Tenancy with Tenant Identifiers for Shared Process Engine](multi-tenancy/tenant-identifier-shared) | All | Multi-Tenancy |
| [Multi-Tenancy with Tenant Identifiers and Shared Process Definitions](multi-tenancy/tenant-identifier-shared-definitions) | Unit Test | Multi-Tenancy |
### Spin Examples
| Name | Container | Keywords |
|------------------------------------------------------------------------------------------------------------------------------------------------|--------------------|------------------------------------|
| [Global Data Format Configuration to Customize JSON serialization](spin/dataformat-configuration-global) | Unit Test | Spin, Configuration |
| [Process-Application-Specific Data Format Configuration to Customize JSON serialization](spin/dataformat-configuration-in-process-application) | Application Server | Spin, Configuration, Shared Engine |
### DMN Examples
| Name | Container | Keywords |
|---------------------------------------------------------------------------------------|-----------|-----------------|
| [Embed Decision Engine - Dish Decision Maker](dmn-engine/dmn-engine-java-main-method) | Jar | DMN, Embed |
| [Decision Requirements Graph(DRG) Example](dmn-engine/dmn-engine-drg) | Jar | DMN, DRG, Embed |
### Process Instance Migration Examples
| Name | Container | Keywords |
|-----------------------------------------------------------------------------------|---------------------------------------|-----------------|
| [Migration on Deployment of New Process Version](migration/migrate-on-deployment) | Application Server with Shared Engine | BPMN, Migration |
### Authentication
| Name | Container | Keywords |
|---------------------------------------------------|----------------------------------------------------------------------------|-------------------------|
| [Basic Authentication](authentication/basic) [^1] | Spring boot with embedded engine, REST API and Basic Authentication filter | Authentication |
### Spring Boot Starter examples
| Name | Container | Keywords |
|-------------------------------------------------------------------|--------------------------------------------------------|---------------------------------------|
| [Plain Camunda Engine](spring-boot-starter/example-simple) | Jar | Spring Boot Starter |
| [Webapps](spring-boot-starter/example-webapp) | Spring boot with embedded engine and Webapps | Spring Boot Starter, Webapps |
| [Webapps EE](spring-boot-starter/example-webapp-ee) | Spring boot with embedded engine and Webapps | Spring Boot Starter, Webapps |
| [REST API](spring-boot-starter/example-web) | Spring boot with embedded engine and Webapps | Spring Boot Starter, REST API |
| [Twitter](spring-boot-starter/example-twitter) | Spring boot with embedded engine and Webapps | Spring Boot Starter, Webapps, Twitter |
| [Camunda Invoice Example](spring-boot-starter/example-invoice) | Spring boot with embedded engine, Webapps and Rest API | Spring Boot Starter, REST API |
| [Autodeployment](spring-boot-starter/example-autodeployment) [^1] | Spring boot with embedded engine and Webapps | Spring Boot Starter |
| [REST API DMN](spring-boot-starter/example-dmn-rest) [^1] | Spring boot with embedded engine and Webapps | Spring Boot Starter, REST API |
### Quarkus Extension Examples
| Name | Container | Keywords |
|-------------------------------------------------------------------|----------------------------------------------------------------------------|-------------------------|
| [Datasource Example](quarkus-extension/datasource-example) | Uber-Jar | Quarkus Extension |
| [Spin Plugin Example](quarkus-extension/spin-plugin-example) | Uber-Jar | Quarkus Extension |
| [Simple REST Example](quarkus-extension/simple-rest-example) [^1] | Uber-Jar | Quarkus Extension |
### External Task Client
| Name | Environment | Keywords |
|------------------------------------------------------------------------------------------------------------------------------|-------------------------------------|-----------------------------------|
| [Order Handling - Java](./clients/java/order-handling) | Java External Task Client | External Task Client, Servicetask |
| [Loan Granting - Java](./clients/java/loan-granting) [^1] | Java External Task Client | External Task Client, Servicetask |
| [Dataformat - Java](./clients/java/dataformat) [^1] | Java External Task Client | External Task Client, Servicetask |
| [Loan Granting - JavaScript](https://github.com/camunda/camunda-external-task-client-js/tree/master/examples/granting-loans) | JavaScript External Task Client | External Task Client, Servicetask |
| [Order Handling - JavaScript](https://github.com/camunda/camunda-external-task-client-js/tree/master/examples/order) | JavaScript External Task Client | External Task Client, Servicetask |
### External Task Client Spring
| Name | Keywords |
|------------------------------------------------------------------------------------------|-----------------------------------|
| [Loan Granting Example](./spring-boot-starter/external-task-client/loan-granting-spring) | External Task Client, Servicetask |
### External Task Client Spring Boot
| Name | Container | Keywords |
|---------------------------------------------------------------------------------------------------------------------------|-----------------------------------------------------|--------------------------------------------------------|
| [Loan Granting w/ REST API & Webapp Example](./spring-boot-starter/external-task-client/loan-granting-spring-boot-webapp) | Spring Boot with embedded Client, REST API & Webapp | External Task Client, Servicetask, Spring Boot Starter |
| [Order Handling Example](./spring-boot-starter/external-task-client/order-handling-spring-boot) [^1] | Spring Boot with embedded Client | External Task Client, Servicetask, Spring Boot Starter |
| [Request Interceptor Example](./spring-boot-starter/external-task-client/request-interceptor-spring-boot) [^1] | Spring Boot with embedded Client | External Task Client, Servicetask, Spring Boot Starter |
### Container Specifics
| Name | Container | Keywords |
|-----------------------------------------------------------------------|-----------|--------------|
| [Jackson Annotation Example for WildFly](wildfly/jackson-annotations) | Wildfly | War, Servlet |
### Testing
| Name | Keywords |
|------------------------------------------------------------------------------------------------------|--------------------------|
| [Assert](testing/assert/job-announcement-publication-process) | Testing, Junit 4, Assert |
| [Assert and JUnit 5](testing/junit5/camunda-bpm-junit-assert/) | Testing, Junit 5, Assert |
| [Assert and Junit 5: configure a custom process engine](testing/junit5/camunda-bpm-junit-use-engine) | Testing, Junit 5, Assert |
## Contributing
Have a look at our [contribution guide](https://github.com/camunda/camunda-bpm-platform/blob/master/CONTRIBUTING.md) for how to contribute to this repository.
## License
The source files in this repository are made available under the [Apache License Version 2.0](./LICENSE).
[^1]: _This example is not actively maintained anymore._
[^2]: _Complete demo applications_
| 0 |
Swagger2Markup/swagger2markup | A Swagger to AsciiDoc or Markdown converter to simplify the generation of an up-to-date RESTful API documentation by combining documentation that’s been hand-written with auto-generated API documentation. | api asciidoc documentation markdown swagger | null | 0 |
deepjavalibrary/djl | An Engine-Agnostic Deep Learning Framework in Java | ai autograd deep-learning deep-neural-networks djl java machine-learning ml mxnet neural-network onnxruntime pytorch tensorflow |




# Deep Java Library (DJL)
## Overview
Deep Java Library (DJL) is an open-source, high-level, engine-agnostic Java framework for deep learning. DJL is designed to be easy to get started with and simple to
use for Java developers. DJL provides a native Java development experience and functions like any other regular Java library.
You don't have to be machine learning/deep learning expert to get started. You can use your existing Java expertise as an on-ramp to learn and use machine learning and deep learning. You can
use your favorite IDE to build, train, and deploy your models. DJL makes it easy to integrate these models with your
Java applications.
Because DJL is deep learning engine agnostic, you don't have to make a choice
between engines when creating your projects. You can switch engines at any
point. To ensure the best performance, DJL also provides automatic CPU/GPU choice based on hardware configuration.
DJL's ergonomic API interface is designed to guide you with best practices to accomplish
deep learning tasks.
The following pseudocode demonstrates running inference:
```java
// Assume user uses a pre-trained model from model zoo, they just need to load it
Criteria<Image, Classifications> criteria =
Criteria.builder()
.optApplication(Application.CV.OBJECT_DETECTION) // find object detection model
.setTypes(Image.class, Classifications.class) // define input and output
.optFilter("backbone", "resnet50") // choose network architecture
.build();
Image img = ImageFactory.getInstance().fromUrl("http://..."); // read image
try (ZooModel<Image, Classifications> model = criteria.loadModel();
Predictor<Image, Classifications> predictor = model.newPredictor()) {
Classifications result = predictor.predict(img);
// get the classification and probability
...
}
```
The following pseudocode demonstrates running training:
```java
// Construct your neural network with built-in blocks
Block block = new Mlp(28 * 28, 10, new int[] {128, 64});
Model model = Model.newInstance("mlp"); // Create an empty model
model.setBlock(block); // set neural network to model
// Get training and validation dataset (MNIST dataset)
Dataset trainingSet = new Mnist.Builder().setUsage(Usage.TRAIN) ... .build();
Dataset validateSet = new Mnist.Builder().setUsage(Usage.TEST) ... .build();
// Setup training configurations, such as Initializer, Optimizer, Loss ...
TrainingConfig config = setupTrainingConfig();
Trainer trainer = model.newTrainer(config);
/*
* Configure input shape based on dataset to initialize the trainer.
* 1st axis is batch axis, we can use 1 for initialization.
* MNIST is 28x28 grayscale image and pre processed into 28 * 28 NDArray.
*/
trainer.initialize(new Shape(1, 28 * 28));
EasyTrain.fit(trainer, epoch, trainingSet, validateSet);
// Save the model
model.save(modelDir, "mlp");
// Close the resources
trainer.close();
model.close();
```
## [Getting Started](docs/quick_start.md)
## Resources
- [Documentation](docs/README.md#documentation)
- [DJL's D2L Book](https://d2l.djl.ai/)
- [JavaDoc API Reference](https://djl.ai/website/javadoc.html)
## Release Notes
* [0.27.0](https://github.com/deepjavalibrary/djl/releases/tag/v0.27.0) ([Code](https://github.com/deepjavalibrary/djl/tree/v0.27.0))
* [0.26.0](https://github.com/deepjavalibrary/djl/releases/tag/v0.26.0) ([Code](https://github.com/deepjavalibrary/djl/tree/v0.26.0))
* [0.25.0](https://github.com/deepjavalibrary/djl/releases/tag/v0.25.0) ([Code](https://github.com/deepjavalibrary/djl/tree/v0.25.0))
* [0.24.0](https://github.com/deepjavalibrary/djl/releases/tag/v0.24.0) ([Code](https://github.com/deepjavalibrary/djl/tree/v0.24.0))
* [0.23.0](https://github.com/deepjavalibrary/djl/releases/tag/v0.23.0) ([Code](https://github.com/deepjavalibrary/djl/tree/v0.23.0))
* [+23 releases](https://github.com/deepjavalibrary/djl/releases)
The release of DJL 0.28.0 is planned for May 2024.
## Building From Source
To build from source, begin by checking out the code.
Once you have checked out the code locally, you can build it as follows using Gradle:
```sh
# for Linux/macOS:
./gradlew build
# for Windows:
gradlew build
```
To increase build speed, you can use the following command to skip unit tests:
```sh
# for Linux/macOS:
./gradlew build -x test
# for Windows:
gradlew build -x test
```
### Importing into eclipse
to import source project into eclipse
```sh
# for Linux/macOS:
./gradlew eclipse
# for Windows:
gradlew eclipse
```
in eclipse
file->import->gradle->existing gradle project
**Note:** please set your workspace text encoding setting to UTF-8
## Community
You can read our guide to [community forums, following DJL, issues, discussions, and RFCs](docs/forums.md) to figure out the best way to share and find content from the DJL community.
Join our [<img src='https://cdn3.iconfinder.com/data/icons/social-media-2169/24/social_media_social_media_logo_slack-512.png' width='20px' /> slack channel](http://tiny.cc/djl_slack) to get in touch with the development team, for questions and discussions.
Follow our [<img src='https://cdn2.iconfinder.com/data/icons/social-media-2285/512/1_Twitter_colored_svg-512.png' width='20px' /> twitter](https://twitter.com/deepjavalibrary) to see updates about new content, features, and releases.
关注我们 [<img src='https://www.iconfinder.com/icons/5060515/download/svg/512' width='20px' /> 知乎专栏](https://zhuanlan.zhihu.com/c_1255493231133417472) 获取DJL最新的内容!
## Useful Links
* [DJL Website](https://djl.ai/)
* [Documentation](https://docs.djl.ai/)
* [DJL Demos](https://docs.djl.ai/docs/demos/index.html)
* [Dive into Deep Learning Book Java version](https://d2l.djl.ai/)
## License
This project is licensed under the [Apache-2.0 License](LICENSE).
| 0 |
danfickle/openhtmltopdf | An HTML to PDF library for the JVM. Based on Flying Saucer and Apache PDF-BOX 2. With SVG image support. Now also with accessible PDF support (WCAG, Section 508, PDF/UA)! | accessibility css html java pdf pdf-generation pdfbox svg | [](https://github.com/danfickle/openhtmltopdf/actions?query=workflow%3Abuild)
# OPEN HTML TO PDF

## OVERVIEW
Open HTML to PDF is a pure-Java library for rendering a reasonable subset of well-formed XML/XHTML (and even some HTML5)
using CSS 2.1 (and later standards) for layout and formatting, outputting to PDF or images.
Use this library to generated nice looking PDF documents. But be aware that you can not throw modern HTML5+ at
this engine and expect a great result. You must special craft the HTML document for this library and
use it's extended CSS feature like [#31](https://github.com/danfickle/openhtmltopdf/pull/31) or
[#32](https://github.com/danfickle/openhtmltopdf/pull/32)
to get good results. Avoid floats near page breaks and use table layouts.
## GETTING STARTED
+ [Integration guide](https://github.com/danfickle/openhtmltopdf/wiki/Integration-Guide) - get maven artifacts and code to get started.
+ [1.0.10 Online Sandbox](https://sandbox.openhtmltopdf.com/) - Now with logs!
+ [Templates for Openhtmltopdf](https://danfickle.github.io/pdf-templates/index.html) - MIT licensed templates that work with this project. Updated 2021-09-21.
+ [Showcase Document - PDF](https://openhtmltopdf.com/showcase.pdf)
+ [Documentation wiki](https://github.com/danfickle/openhtmltopdf/wiki)
+ [Template Author Guide - PDF - DEPRECATED - Prefer wiki](https://openhtmltopdf.com/template-guide.pdf) - Moving info to wiki
+ [Sample Project - Pretty Resume Generator](https://github.com/danfickle/pretty-resume)
## DIFFERENCES WITH FLYING SAUCER
+ Uses the well-maintained and open-source (LGPL compatible) PDFBOX as PDF library, rather than iText.
+ Proper support for generating accessible PDFs (Section 508, PDF/UA, WCAG 2.0).
+ Proper support for generating PDF/A standards compliant PDFs.
+ New, faster renderer means this project can be several times faster for very large documents.
+ Better support for CSS3 transforms.
+ Automatic visual regression testing of PDFs, with many end-to-end tests.
+ Ability to insert pages for cut-off content.
+ Built-in plugins for SVG and MathML.
+ Font fallback support.
+ Limited support for RTL and bi-directional documents.
+ On the negative side, no support for OpenType fonts.
+ Footnote support.
+ Much more. See changelog below.
## LICENSE
Open HTML to PDF is distributed under the LGPL. Open HTML to PDF itself is licensed
under the GNU Lesser General Public License, version 2.1 or later, available at
http://www.gnu.org/copyleft/lesser.html. You can use Open HTML to PDF in any
way and for any purpose you want as long as you respect the terms of the
license. A copy of the LGPL license is included as license-lgpl-2.1.txt or license-lgpl-3.txt
in our distributions and in our source tree.
An exception to this is the pdf-a testing module, which is licensed under the GPL. This module is not distributed to Maven Central
and is for testing only.
Open HTML to PDF uses a couple of FOSS packages to get the job done. A list
of these can be found in the [dependency graph](https://github.com/danfickle/openhtmltopdf/network/dependencies).
## CREDITS
Open HTML to PDF is based on [Flying-saucer](https://github.com/flyingsaucerproject/flyingsaucer). Credit goes to the contributors of that project. Code will also be used from [neoFlyingSaucer](https://github.com/danfickle/neoflyingsaucer)
## FAQ
+ OPEN HTML TO PDF is tested with OpenJDK 8, 11 and 17 (early access). It requires at least Java 8 to run.
+ No, you can not use it on Android.
+ You should be able to use it on Google App Engine (Java 8 or greater environment). [Let us know your experience](https://github.com/danfickle/openhtmltopdf/issues/179).
+ <s>Flowing columns are not implemented.</s> Implemented in RC12.
+ No, it's not a web browser. Specifically, it does not run javascript or implement many modern standards such as flex and grid layout.
## TEST CASES
Test cases, failing or working are welcome, please place them
in ````/openhtmltopdf-examples/src/main/resources/testcases/````
and run them
from ````/openhtmltopdf-examples/src/main/java/com/openhtmltopdf/testcases/TestcaseRunner.java````.
## CHANGELOG
### head - 1.0.11-SNAPSHOT
+ See commit log.
### 1.0.10 (2021-September-13)
**NOTE**: After this release the old slow renderer will be deleted. Fast mode has been the default (since 1.0.5) so you only have to check your code if you are calling the `useSlowMode` method which will be removed.
+ [#551](https://github.com/danfickle/openhtmltopdf/issues/551) **SECURITY** Fix near-infinite loop for very deeply nested content with `page-break-inside: avoid` constraint. Thanks for persisting @swillis12 and debugging @syjer.
+ [#729](https://github.com/danfickle/openhtmltopdf/issues/729) **SECURITY** Upgrade xmlgraphics-commons (used in SVG rendering) to avoid CVE. Thanks @electrofLy.
+ [#711](https://github.com/danfickle/openhtmltopdf/pull/711) Footnote support (beta). See [footnote documentation on wiki](https://github.com/danfickle/openhtmltopdf/wiki/Footnotes). Thanks for requesting @a-leithner and @slumki.
+ [#761](https://github.com/danfickle/openhtmltopdf/pull/761) CSS property to disable bevels on borders to prevent ugly anti-aliasing effects, especially on table cells. See [-fs-border-rendering property on wiki](https://github.com/danfickle/openhtmltopdf/wiki/Custom-CSS-properties#-fs-border-rendering-no-bevel). Thanks for providing sample @gandboy91.
+ [#103](https://github.com/danfickle/openhtmltopdf/issues/103) Output exception class name and message by default for log messages with an associated exception.
+ #711 (mixed) Better boxing for `::before` and `::after` content. Should now be able to define a border around pseudo content correctly.
+ [#738](https://github.com/danfickle/openhtmltopdf/issues/738) Support for additional elements in PDF/UA including art, part, sect, section, caption and blockquote. Thanks @AndreasJacobsen.
+ [#736](https://github.com/danfickle/openhtmltopdf/issues/736) New example of using a dom mutator to implement unsupported content such as font tag attributes. Thanks for requesting @mgabhishek06kodur.
+ [#707](https://github.com/danfickle/openhtmltopdf/issues/707) Fix regression where PDF/UA documents that weren't also PDF/A compliant were missing Dublin Core metadata. Thanks @mgm-rwagner, @syjer.
+ [#732](https://github.com/danfickle/openhtmltopdf/issues/732) Allow `table` element to be positioned. Thanks @fcorneli.
+ [#727](https://github.com/danfickle/openhtmltopdf/pull/727) Allow the use of an initial page number for `page` and `pages` counters. Thanks for PR @fanthos.
### 1.0.9 (2021-June-18)
**SECURITY RELEASE**: This release was brought forward due to security releases of the PDFBOX and Batik dependencies.
+ [#722](https://github.com/danfickle/openhtmltopdf/pull/722) Upgrade PDFBOX (to 2.0.24) - avoids CVEs in earlier versions and PDFBoxGraphics2D. Thanks a lot @rototor.
+ [#678](https://github.com/danfickle/openhtmltopdf/pull/678) Upgrade Batik Version to 1.14 (CVE-2020-11987) - Again it is strongly advised to avoid untrusted SVG and XML. Thanks @rototor.
+ [#716](https://github.com/danfickle/openhtmltopdf/pull/716) Replace rogue `println` calls with log calls. Thanks @syjer for PR, @tfo for reporting.
+ [#708](https://github.com/danfickle/openhtmltopdf/pull/708) Allow `shape-rendering` SVG CSS property. Thanks @syjer for PR, @RAlfoeldi for reporting.
+ [#703](https://github.com/danfickle/openhtmltopdf/pull/703) Remove calls to deprecated method calls in JRE standard library. May change XML reader class. Implemented by @danfickle.
+ [#702](https://github.com/danfickle/openhtmltopdf/pull/702) Set timeouts for default HTTP/HTTPS handlers. Thanks for reporting @gengzi.
+ [162228](https://github.com/danfickle/openhtmltopdf/commit/16222810df1cc40dba8bfa1465111b96841bb3b5) Put links to raster images in SVGs through the URL resolver.
+ [#694](https://github.com/danfickle/openhtmltopdf/issues/694) Fix incorrect B3 paper size. Thanks @lfintalan for reporting with line number!
+ [ab48fd](https://github.com/danfickle/openhtmltopdf/commit/ab48fd0d7236ad3129f3393fcb0ebc7df6c5973f) Do not log a missing font more than once.
NOTE: PDFBOX CVEs relate to the loading of untrusted PDFs in PDFBOX and thus this project is not directly affected. However, it is not a good idea to have CVEs on your classpath.
### 1.0.8 (2021-March-22)
**SECURITY RELEASE**
+ [#675](https://github.com/danfickle/openhtmltopdf/issues/675) Update PDFBOX to 2.0.23 to avoid CVEs. Thanks for reporting @Samuel3.
NOTE: These CVEs relate to the loading of untrusted PDFs in PDFBOX and thus this project is not directly affected. However, it is not a good idea to have CVEs on your classpath.
### 1.0.7 (2021-March-19)
+ [#650](https://github.com/danfickle/openhtmltopdf/pull/650) Support for multiple background images on the one element. Thanks for requesting @baedorf.
+ [#669](https://github.com/danfickle/openhtmltopdf/pull/669) Support fallback fonts. Thanks for requesting @asu2 and assisting @draco1023.
+ [#640](https://github.com/danfickle/openhtmltopdf/pull/640) Implement file embeds via the download attribute on links. Thanks for original PR @syjer and for requesting @lindamarieb and @vader.
+ [#666](https://github.com/danfickle/openhtmltopdf/pull/666) API to get the bottom-most y position of rendered content to be able to position follow on content with other tools. Thanks for extensive reviewing of PR @stechio and for request by @DSW-AK.
+ [#664](https://github.com/danfickle/openhtmltopdf/pull/664) Improved support for PDF/A and PDF/UA standards. Thanks for PR @qligier.
+ [#653](https://github.com/danfickle/openhtmltopdf/pull/653) Fix for inline-block elements with a z-index or transform were being output twice. Thanks for reporting @hannes123bsi.
+ [#655](https://github.com/danfickle/openhtmltopdf/pull/655) Correct layout of ordered lists in RTL direction. Thanks for PR @johnnyaug.
+ [#658](https://github.com/danfickle/openhtmltopdf/pull/658) Implement `target-text` function for `content` property. Thanks for PR @BenjaminVega.
+ [#647](https://github.com/danfickle/openhtmltopdf/pull/647) Fix race condition in setting up logger in multi-threaded environments. Thanks for PR @syjer.
+ [#638](https://github.com/danfickle/openhtmltopdf/pull/638) Ability to plug-in external resource control based on resource type and url. Thanks for original PR @syjer.
+ [#628](https://github.com/danfickle/openhtmltopdf/pull/628) Use enhanced image embedding methods from PDF-BOX. Thanks for PR @rototor and your work in PDF-BOX implementing this.
+ [#627](https://github.com/danfickle/openhtmltopdf/pull/627) Fix regression where a null font style was causing NPE. Thaks for PR @rototor.
+ [#338](https://github.com/danfickle/openhtmltopdf/issues/338) Implement read-only radio button group. Thanks for investigating, reporting and patience @ThoSchCon, @aleks-shbln, @dmitry-weirdo, @syjer and @paulito-bandito.
### 1.0.6 (2020-December-22)
**IMPORTANT:** [#615](https://github.com/danfickle/openhtmltopdf/issues/615) This is a bug fix release for an endless loop issue when using break-word with floating elements with a top/bottom margin.
+ [#624](https://github.com/danfickle/openhtmltopdf/pull/624) Update PDFBOX to 2.0.22 and pdfbox-graphics2d to 0.30. Thanks @rototor.
+ [#467](https://github.com/danfickle/openhtmltopdf/issues/467) Prevent possibility of CSS import loop.
+ [#621](https://github.com/danfickle/openhtmltopdf/pull/621) Allow spaces in data uris. Thanks @syjer.
### 1.0.5 (2020-November-30)
**SECURITY:** [#609](https://github.com/danfickle/openhtmltopdf/pull/609) Updates Apache Batik SVG renderer to latest version to avoid security issue. If you are using this project to render untrusted SVGs (advised against), you should update immediately. Thanks a lot @halvorbmundal.
**IMPORTANT:** The fast renderer is now the default in preparation of removing the old slow renderer. To temporarily use the slow renderer, you can call the deprecated method `builder.useSlowMode()` (PDF output only).
**IMPORTANT:** [#543](https://github.com/danfickle/openhtmltopdf/issues/543) This version stays on PDFBOX version 2.0.20 due to a bug with non-breaking spaces in version 2.0.21. Please make sure version 2.0.21 is not on your classpath. This bug has been fixed in the upcoming 2.0.22.
+ [#544](https://github.com/danfickle/openhtmltopdf/pull/544) Code to create a website for pre-canned PDF templates in thymeleaf and raw XHTML format. Check out the [template website](https://danfickle.github.io/pdf-templates/index.html) to preview templates.
+ [#533](https://github.com/danfickle/openhtmltopdf/pull/533) Barcode plugin. Very useful PR supplied by @syjer. [Barcode plugin docs](https://github.com/danfickle/openhtmltopdf/wiki/Plugins:-1D-2D-Barcode-with-ZXing).
+ [#521](https://github.com/danfickle/openhtmltopdf/pull/521) Move Java2D image output to fast renderer and general improvements. [Java2D image output docs](https://github.com/danfickle/openhtmltopdf/wiki/Java2D-Image-Output).
+ [9ffd0e](https://github.com/danfickle/openhtmltopdf/commit/9ffd0e4ad4a14e06a8c3921c3849f6bef83cef74) [#568](https://github.com/danfickle/openhtmltopdf/pull/568) Filter out problematic characters that are visible in some fonts but should not be such as soft-hyphen. Thanks @StephanSchrader.
+ [#587](https://github.com/danfickle/openhtmltopdf/pull/587) Fix for white-space: nowrap cutting off instead of wrapping. Thanks @vipcxj for finally fixing via PR.
+ [#577](https://github.com/danfickle/openhtmltopdf/pull/577) Add foreground PDF drawer plugin (useful especially for watermarks). Thanks @rototor for PR and @sillen102 for persisting.
+ [#566](https://github.com/danfickle/openhtmltopdf/issues/566) Rename `baseUri` arg to `baseDocumentUri` and improve javadoc to avoid confusion. Thanks for reporting @NehalDamania.
+ [801780](https://github.com/danfickle/openhtmltopdf/commit/801780ba03537d004b3423485a3ac7692a09bb95) Update junit test dependency to 4.13.1 to avoid security scanner warnings (the specific security problem did not impact this library).
+ [#553](https://github.com/danfickle/openhtmltopdf/issues/553) Fix for ContentLimitContainer causing NPEs when negative margins are used. Thanks for reporting @adilxoxo.
+ [#552](https://github.com/danfickle/openhtmltopdf/pull/552) Optimize the log formatter for j.u.l logging. Thanks for the impressive PR @syjer.
+ [#542](https://github.com/danfickle/openhtmltopdf/pull/542) Improve list-decoration placement. Thanks for PR @syjer and reporting @mndzielski.
+ [#458](https://github.com/danfickle/openhtmltopdf/issues/458) Fix for list-decorations being output (clipped) in page margin area.
+ [#525](https://github.com/danfickle/openhtmltopdf/pull/525) Remove unused schema/DTDs. Significantly reduces size of jar. Thanks for PR @syjer.
+ [#592](https://github.com/danfickle/openhtmltopdf/issues/592) Allow unit (px, cm, em, etc) values in the width/height attributes of linked SVG images. Thanks @DanielWulfert.
+ [#594](https://github.com/danfickle/openhtmltopdf/issues/594) [#458](https://github.com/danfickle/openhtmltopdf/issues/458) Fix for more repeating content and PDF/UA crash. Thanks @ThomHurks, @fungc.
+ [#599](https://github.com/danfickle/openhtmltopdf/issues/599) Fix RuntimeException ocurring on InlineText.setSubstring. Thanks @LAlves91.
+ [#605](https://github.com/danfickle/openhtmltopdf/issues/605) Fix to make justification work with surrogate pairs. Thanks @EmanuelCozariz.
+ [#601](https://github.com/danfickle/openhtmltopdf/pull/601) Move CI to Github actions. Thanks @syjer.
+ [#597](https://github.com/danfickle/openhtmltopdf/pull/597) Generalize data uri support. Thanks @syjer, @Leostat86.
+ [#613](https://github.com/danfickle/openhtmltopdf/pull/613) Allow adding fonts for SVG, MathML as files instead of input streams to avoid JDK bug. Thanks @syjer, @sureshkumar-ramalingam, @olayinkasf.
### 1.0.4 (2020-July-25)
+ [b88538](https://github.com/danfickle/openhtmltopdf/commit/b8853841b44d1e689d9bb3510a36633485fe21ab) Fix for endless loop when using `word-wrap: break-word`. Thanks for reporting, testing and investigating @swarl. Thanks for tests and debugging @rototor and @syjer.
+ [#492](https://github.com/danfickle/openhtmltopdf/pull/492) Lots of testing of the line-breaking algorithm to avoid future endless loops. By @danfickle.
+ [#515](https://github.com/danfickle/openhtmltopdf/pull/515) Pass document CSS styles applying to SVG element to SVG implementation. Thanks for requesting and contributing @amckain92.
+ [#514](https://github.com/danfickle/openhtmltopdf/issues/514) FIX: Correctly position boxes when justifying rtl lines. Thanks for reporting and testing @lzhy1101.
+ [#512](https://github.com/danfickle/openhtmltopdf/pull/512) [#507](https://github.com/danfickle/openhtmltopdf/pull/507) [#502](https://github.com/danfickle/openhtmltopdf/pull/502) Cleanup code including deleting unused code, generics, etc. Thanks for PRs @syjer.
+ [#489](https://github.com/danfickle/openhtmltopdf/pull/489) Extensive overhaul of logging including per run diagnostic consumer. Huge thanks @syjer, a lot of work in this PR. See [logging page on wiki](https://github.com/danfickle/openhtmltopdf/wiki/Logging) for more info.
+ [#501](https://github.com/danfickle/openhtmltopdf/pull/501) Upgrade PDFBOX to 2.0.20 and PDFBox-Graphics2D to 0.26. Thanks for PR @rototor.
+ [#490](https://github.com/danfickle/openhtmltopdf/pull/490) Fix for NPE when decoding image data url fails. Thanks for PR @syjer and reporting @AlexisCothenet.
+ [#516](https://github.com/danfickle/openhtmltopdf/pull/516) Add OSGI bundle metadata to MANIFEST.MFs. Thanks for requesting and investigating @zspitzer.
### 1.0.3 (2020-May-25)
+ **IMPORTANT**: This release contains fixes for two bugs that may result in endless loops/denial of service when using `word-wrap: break-word`. If you are using this feature, please upgrade promptly.
+ [#483](https://github.com/danfickle/openhtmltopdf/pull/483) Fix for endless loop bug with `word-wrap: break-word` and soft hyphens. Thanks @rototor for PR, @syjer for analysis and @swarl for reporting.
+ [#466](https://github.com/danfickle/openhtmltopdf/issues/466) Fix for endless loop bug with `word-wrap: break-word` and zero width boxes. Thanks @syjer for analysis and @AlexisCothenet for reporting.
+ [#486](https://github.com/danfickle/openhtmltopdf/pull/486) SVG plugin can now provide a list of allowed protocols for external resources and any configured uri resolver/stream handlers will be used. Thanks @syjer for PR and @ieugen for reporting.
+ [#480](https://github.com/danfickle/openhtmltopdf/pull/480) Fix for link shapes being returned from custom object drawers. Thanks @rototor for PR and @hbergmey for reporting.
+ [#485](https://github.com/danfickle/openhtmltopdf/pull/485) Implement support for SVG data uris. Thanks @syjer for PR and @adrianrodfer for reporting.
+ [#470](https://github.com/danfickle/openhtmltopdf/pull/470) Allow `mailto:` links or any other valid link. Thanks @syjer for PR and @mndzielski for reporting.
+ [#464](https://github.com/danfickle/openhtmltopdf/issues/464) Honor the `direction` CSS property. Thanks @AnanasPizza for reporting.
+ [#460](https://github.com/danfickle/openhtmltopdf/pull/460) Change thrown exception class to more specific `IOException`. Thanks for PR @leonorader.
+ [#459](https://github.com/danfickle/openhtmltopdf/issues/459) Implement the `rem` CSS unit. Thanks to @leonorader for reporting.
+ [#211](https://github.com/danfickle/openhtmltopdf/issues/211) Images can now be used in the CSS `content` property. Thanks for requesting @Kuhlware.
+ [#445](https://github.com/danfickle/openhtmltopdf/issues/445) Fix for not picking up attribute values in Jsoup converted documents. Thanks for reporting @testinfected.
+ [#450](https://github.com/danfickle/openhtmltopdf/pull/450) Java2D output only: Ability to add fonts via code. Also environment fonts will no longer be used by default. To use environment fonts: `builder.useEnvironmentFonts(true)`.
### 1.0.2 (2020-February-25)
+ [SECURITY](https://github.com/danfickle/openhtmltopdf/commit/82bdedaf341ef3368dcebbb700a60ef870ab89b5) Removed Log4J 1.x adaptor as it had CVE-2019-17571 with no updated version available.
+ [#448](https://github.com/danfickle/openhtmltopdf/pull/448) Implement `linear-gradient` support for `background-image` property. By @danfickle. Requested by @rja907.
+ [#429](https://github.com/danfickle/openhtmltopdf/issues/429) Major overhaul of `word-wrap: break-word`. Now a word will not be broken unless it is too big for a line by itself. By @danfickle. Thanks for reporting and testing @mndzielski.
+ [#433](https://github.com/danfickle/openhtmltopdf/issues/433) Do not justify lines ending with `<br/>` tag. Thanks for reporting @fcorneli.
+ [#440](https://github.com/danfickle/openhtmltopdf/issues/440) Remove trailing white space for right aligned text to avoid jagged appearance. Thanks for reporting @AnanasPizza.
+ [#446](https://github.com/danfickle/openhtmltopdf/issues/446) Look for lang attribute on ancestor elements when using `lang()` selector. Thanks for reporting and tracking down the bug @fungc.
+ [#430](https://github.com/danfickle/openhtmltopdf/pull/430) Use relative path to license in source jars instead of absolute path. Thanks for reporting @gabro and fixing via PR @syjer.
+ [#417](https://github.com/danfickle/openhtmltopdf/issues/417) Keep aspect ratio of images with width/height properties as well as min/max width/height properties. Thanks for reporting and basis for fix @swarl.
+ [#423](https://github.com/danfickle/openhtmltopdf/pull/423) Allow multiple font sources to be specified with `format` tags. Only use `format(truetype)`. Thanks for requesting @MichaelZaleskovsky and basis for implementation @syjer.
+ [#415](https://github.com/danfickle/openhtmltopdf/pull/415) Avoid class cast exception if user tries to float table cell. Thanks for reporting @dmartineau99 and PR @syjer.
+ [#421](https://github.com/danfickle/openhtmltopdf/pull/421) Avoid NPE when justified text is mixed with unjustifiable content. Thanks for reporting @Megingjard and PR @syjer.
+ Updated PDFBOX 2.0.17 to 2.0.19.
### 1.0.1 (2019-November-18)
+ [#413](https://github.com/danfickle/openhtmltopdf/pull/413) Handle form problems such as no name on input element without throwing a NPE. Thanks @syjer for PR and @mmatecki for reporting.
+ [#412](https://github.com/danfickle/openhtmltopdf/pull/412) Add HTML block level elements usch as `section` to default CSS. Thanks @syjer.
+ [#339](https://github.com/danfickle/openhtmltopdf/issues/339) Remove the JSoup to DOM converter module. Thanks @kewilson.
+ [0cd098](https://github.com/danfickle/openhtmltopdf/commit/0cd09893af364c184403497ed0a9cc4df8f3073a) Fix for letter-spacing support on last line of block with trailing space. Also performance improvements and refactoring. By @danfickle.
+ [#410](https://github.com/danfickle/openhtmltopdf/pull/410) Fix for wrong bold setting on list item counters. Thanks @syjer for PR fix (and test!) and @acieplinski for reporting.
+ [Wiki](https://github.com/danfickle/openhtmltopdf/wiki/Custom-CSS-properties#-fs-max-justification-inter-char-and--fs-max-justification-inter-word) Configurable text justification settings as part of a justification overhaul that also allows more space to be used inter-char when there are no spaces on the line. By @danfickle. Commits listed in #403.
+ [#403](https://github.com/danfickle/openhtmltopdf/issues/403) Soft hyphen support. Soft hyphens are now replaced with hard hyphens when used as line ending character. Thanks @sbrunecker.
+ [#408](https://github.com/danfickle/openhtmltopdf/issues/408) Fix for bookmarks not working with HTML5 parsers such as JSoup. Thanks @syjer for investigating and fixing and @Milchreis for reporting.
+ [#404](https://github.com/danfickle/openhtmltopdf/issues/404) Upgrade Batik to 1.12 and xmlgraphics-common to 2.4 (both used in SVG module) to avoid CVE in one or both. Thanks @avoiculet.
+ [#396](https://github.com/danfickle/openhtmltopdf/issues/396) Much faster rendering of boxes using border-radius properties. Thanks @mndzielski.
+ [#400](https://github.com/danfickle/openhtmltopdf/pull/400) Support for `lang` and `title` attrbiutes and `abbr` tag for accessible PDFs. Thanks @Ignaciort91.
+ [#394](https://github.com/danfickle/openhtmltopdf/pull/394), [#395](https://github.com/danfickle/openhtmltopdf/pull/395) Upgrade PDFBOX to 2.0.17 and pdfbox-graphics2d to 0.25. Thanks @cristan, @rototor.
+ [#384](https://github.com/danfickle/openhtmltopdf/pull/384) Allow user to provide PDFont supplier. Thanks @DSW-PS.
+ [#373](https://github.com/danfickle/openhtmltopdf/pull/373) Fix regression where both max-width and max-height are provided for images with certain aspect ratios. Thanks @rototor.
+ [#380](https://github.com/danfickle/openhtmltopdf/pull/380) Much better support for flowing columns including explicit column breaks, floating content, block level nested content. By @danfickle.
### 1.0.0 (2019-July-23)
+ [#372](https://github.com/danfickle/openhtmltopdf/pull/372) Much improved sizing support for `img`, `svg` and `math` elements.
+ [#344](https://github.com/danfickle/openhtmltopdf/issues/344) Use PDFs in `img` tag: `<img src="some.pdf" page="1" alt="Some alt text" />`.
### OLDER RELEASES
[View CHANGELOG.md](CHANGELOG.md).
| 0 |
JessYanCoding/RetrofitUrlManager | 🔮 Let Retrofit support multiple baseUrl and can be change the baseUrl at runtime (以最简洁的 Api 让 Retrofit 同时支持多个 BaseUrl 以及动态改变 BaseUrl). | arms baseurl baseurl-domain mvparms okhttp okhttp3 retrofit retrofit2 | # RetrofitUrlManager
[  ](https://bintray.com/jessyancoding/maven/retrofit-url-manager/1.4.0/link)
[  ](https://travis-ci.org/JessYanCoding/RetrofitUrlManager)
[  ](https://android-arsenal.com/details/1/6007)
[  ](https://developer.android.com/about/versions/android-2.3.html)
[  ](http://www.apache.org/licenses/LICENSE-2.0)
[  ](https://www.jianshu.com/u/1d0c0bc634db)
[  ](https://shang.qq.com/wpa/qunwpa?idkey=7e59e59145e6c7c68932ace10f52790636451f01d1ecadb6a652b1df234df753)
## Let Retrofit support multiple baseUrl and can be change the baseUrl at runtime.
[中文说明](README-zh.md)
## Overview

## Notice
[**Framework analysis 1**](http://www.jianshu.com/p/2919bdb8d09a)
[**Framework analysis 2**](https://www.jianshu.com/p/35a8959c2f86)
[**More complete sample**](https://github.com/JessYanCoding/ArmsComponent)
## Download
``` gradle
implementation 'me.jessyan:retrofit-url-manager:1.4.0'
```
## Usage
### Initialize
``` java
// When building OkHttpClient, the OkHttpClient.Builder() is passed to the with() method to initialize the configuration
OkHttpClient = RetrofitUrlManager.getInstance().with(new OkHttpClient.Builder())
.build();
```
### Step 1
``` java
public interface ApiService {
@Headers({"Domain-Name: douban"}) // Add the Domain-Name header
@GET("/v2/book/{id}")
Observable<ResponseBody> getBook(@Path("id") int id);
}
```
### Step 2
``` java
// You can change BaseUrl at any time while App is running (The interface that declared the Domain-Name header)
RetrofitUrlManager.getInstance().putDomain("douban", "https://api.douban.com");
```
### If you want to change the global BaseUrl:
```java
// BaseUrl configured in the Domain-Name header will override BaseUrl in the global setting
RetrofitUrlManager.getInstance().setGlobalDomain("your BaseUrl");
```
## About Me
* **Email**: <jess.yan.effort@gmail.com>
* **Home**: <http://jessyan.me>
* **掘金**: <https://juejin.im/user/57a9dbd9165abd0061714613>
* **简书**: <https://www.jianshu.com/u/1d0c0bc634db>
## License
```
Copyright 2017, jessyan
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
```
| 0 |
google/j2cl | Java to Closure JavaScript transpiler | compiler gwt j2cl java javascript transpiler wasm wasmgc | # [J2CL](http://j2cl.io) · [](https://github.com/google/j2cl/actions/workflows/ci.yaml)
Seamless Java in JavaScript applications that tightly optimizes with
[Closure Compiler](https://github.com/google/closure-compiler)
---
J2CL is a powerful, simple and lightweight transpiler from Java to Closure style
JavaScript.
* **Get the best out of Java and JavaScript.** You no longer need to choose between
the two or lock into a specific framework or a language. Choose the right language
at the right place and hire the best talent for the job.
* **Get it correct the first time.** The robust run-time type checking based on
the strong Java type system combined with the advanced cross language type checks
catches your mistakes early on.
* **Provides massive code reuse.** J2CL closely follows the Java language
[semantics](docs/limitations.md). This reduces surprises, enables reuse across
different platforms and brings most popular Java libraries into your toolkit
including [Guava](https://github.com/google/guava), [Dagger](https://dagger.dev/)
and [AutoValue](https://github.com/google/auto/tree/master/value).
* **Modern, fresh and blazing fast.** Powered by [Bazel](https://bazel.build/),
J2CL provides a fast and modern development experience that will make you smile
and keep you productive.
* **Road tested and trusted.** J2CL is the underlying technology of the most
advanced GSuite apps developed by Google including GMail, Inbox, Docs, Slides
and Calendar.
Guides
------
- [Getting Started](docs/getting-started.md)
- [Getting Started for Wasm](docs/getting-started-j2wasm.md)
- [JsInterop Cookbook](docs/jsinterop-by-example.md)
- [J2CL Best Practices](docs/best-practices.md)
- [Emulation Limitations](docs/limitations.md)
- [Bazel Tutorial](https://docs.bazel.build/versions/master/tutorial/java.html)
- [Bazel Best Practices](https://docs.bazel.build/versions/master/best-practices.html)
Get Support
------
- Please subscribe to [J2CL announce](http://groups.google.com/forum/#!forum/j2cl-announce) for announcements (low traffic).
- Please report [bugs](https://github.com/google/j2cl/issues/new?template=bug_report.md&labels=bug)
or file [feature requests](https://github.com/google/j2cl/issues/new?template=feature_request.md&labels=enhancement)
via [issue tracker](https://github.com/google/j2cl/issues).
- For other questions please use the [discussions](https://github.com/google/j2cl/discussions/new?category=q-a).
Caveat Emptor
-------------
J2CL is production ready and actively used by many of Google's products, but the
process of adapting workflows and tooling for the open-source version is not yet
finalized.
Wasm support is fully working but should be considered as work-in-progress since
the related parts of the spec is not finalized yet. We are working closely with
W3C and V8 team to finalize it and make it available in all browsers.
You can see what we are working on [here](https://github.com/google/j2cl/issues/93).
Last, the workflow is **not** yet supported in Windows. You can contribute to
make this a reality. Coordinate and follow the progress of this effort
[here](https://github.com/google/j2cl/issues/9).
For developers that want to use Windows as their platform we recommend
installing under WSL (Windows Subsystem for Linux).
Stay tuned!
J2CL vs. GWT?
---
In early 2015, Google GWT team made a difficult but necessary decision to work
on a new alternative product to enable Java for the Web.
It was mostly due to changing trends in the web ecosystem and our new internal
customers who were looking at Java on the Web not as an isolated ecosystem but
an integral part of their larger stack. It required a completely new vision
to create tools from the ground up, that are tightly integrated with the rest of
the ecosystem. A modern architecture, that is reliable, fast and provides a
quick iteration cycle.
There was no practical way to achieve those goals completely incrementally out
of GWT. We started from scratch using everything we learned from working on GWT
over the years. In the meantime, we kept GWT steering committee members in the
loop and gave contributors very early access so they could decide to build the
next version of GWT on J2CL.
The strategy has now evolved GWT3 to an SDK focused on libraries and enterprise
tooling which was one of the strongest points of GWT all along.
We think that such separation of concerns is crucial part of the success of the
both projects and will provide the best results for the open source community.
Contributing
------------
Read how to [contribute to J2CL](CONTRIBUTING.md).
Licensing
---------
Please refer to [the license file](LICENSE).
Disclaimers
-----------
J2CL is not an official Google product and is currently in 'alpha' release for developer preview.
| 0 |
Zo3i/frpMgr | Frp快速配置面板 | null | [README](README.md) | [中文文档](README_zh.md)
---
### Function:
- help you to Configuration frp client and server.
- install frp server on the web.
- look up each server connect status.
### Install Tutorial
```shell
wget -O - https://raw.githubusercontent.com/Zo3i/OCS/master/docker/docker-all2.sh | sh
wget -O - https://raw.githubusercontent.com/Zo3i/frpMgr/master/web/src/main/docker/final/run.sh | sh
```
just run the code above on your server;
**Tip:the code just test on Debian9 CentOs7 and Ubantu18**
- **how to access**: your server ip:8999/frp
- **default account and password**: admin/12345678
- **log information** :1. docker ps 2. docker logs -f --tail 10 your java container ID
### How to Use:
- Resolve `*.xxx.com` to the frps server's IP. This is usually called a Wildcard DNS record.
- Configuration the server.
- install server online.
- Configuration the client.
- run open.bat.
## Example Usage
- Resolve `*.xxx.com` to the frps server's IP

---
- Configuration the server.

---

- input your remote server's password and wait minutes .(**don't worry about your password disclosure. it just varify your server**)
- after you install and run the frp server. you can check out or restart the server
```shell
service frps status
service frps stop
service frps restart
```
---
## Star History
[](https://star-history.com/#Zo3i/frpMgr&Timeline)
| 0 |
bonigarcia/webdrivermanager | Automated driver management and other helper features for Selenium WebDriver in Java | chromedriver docker geckodriver java java-agent maven selenium selenium-webdriver | [](https://search.maven.org/search?q=g:io.github.bonigarcia%20a:webdrivermanager)
[](https://github.com/bonigarcia/webdrivermanager/actions)
[](https://sonarcloud.io/summary/new_code?id=io.github.bonigarcia%3Awebdrivermanager)
[](https://codecov.io/gh/bonigarcia/webdrivermanager)
[](https://www.oracle.com/java/technologies/javase-downloads.html)
[](https://www.apache.org/licenses/LICENSE-2.0)
[](#backers)
[](#sponsors)
[](https://stackoverflow.com/questions/tagged/webdrivermanager-java)
[](https://twitter.com/boni_gg)
# [![][Logo]][WebDriverManager]
[WebDriverManager] is an open-source Java library that carries out the management (i.e., download, setup, and maintenance) of the drivers required by [Selenium WebDriver] (e.g., chromedriver, geckodriver, msedgedriver, etc.) in a fully automated manner. In addition, WebDriverManager provides other relevant features, such as the capability to discover browsers installed in the local system, building WebDriver objects (such as `ChromeDriver`, `FirefoxDriver`, `EdgeDriver`, etc.), and running browsers in Docker containers seamlessly.
## Documentation
As of version 5, the documentation of WebDriverManager has moved [here][WebDriverManager]. This site contains all the features, examples, configuration, and advanced capabilities of WebDriverManager.
## Driver Management
The primary use of WebDriverManager is the automation of driver management. For using this feature, you need to select a given manager in the WebDriverManager API (e.g., `chromedriver()` for Chrome) and invoke the method `setup()`. The following example shows the skeleton of a test case using [JUnit 5], [Selenium WebDriver], and [WebDriverManager].
```java
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.BeforeAll;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import io.github.bonigarcia.wdm.WebDriverManager;
class ChromeTest {
WebDriver driver;
@BeforeAll
static void setupAll() {
WebDriverManager.chromedriver().setup();
}
@BeforeEach
void setup() {
driver = new ChromeDriver();
}
@AfterEach
void teardown() {
driver.quit();
}
@Test
void test() {
// Your test logic here
}
}
```
Alternatively, you can use the method `create()` to manage automatically the driver and instantiate the `WebDriver` object in a single line. For instance, as follows:
```java
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import io.github.bonigarcia.wdm.WebDriverManager;
class ChromeCreateTest {
WebDriver driver;
@BeforeEach
void setup() {
driver = WebDriverManager.chromedriver().create();
}
@AfterEach
void teardown() {
driver.quit();
}
@Test
void test() {
// Your test logic here
}
}
```
For further information about the driver resolution algorithm implemented by WebDriverManager and configuration capabilities, read the [documentation][WebDriverManager].
## Browsers in Docker
Another relevant new feature available in WebDriverManager 5 is the ability to create browsers in [Docker] containers out of the box. The requirement to use this feature is to have installed a [Docker Engine] in the machine running the tests. To use it, we need to invoke the method `browserInDocker()` in conjunction with `create()` of a given manager. This way, WebDriverManager pulls the image from [Docker Hub], starts the container, and instantiates the WebDriver object to use it. The following test shows a simple example using Chrome in Docker. This example also enables the recording of the browser session and remote access using [noVNC]:
```java
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.openqa.selenium.WebDriver;
import io.github.bonigarcia.wdm.WebDriverManager;
class DockerChromeVncTest {
WebDriver driver;
WebDriverManager wdm = WebDriverManager.chromedriver().browserInDocker()
.enableVnc().enableRecording();
@BeforeEach
void setup() {
driver = wdm.create();
}
@AfterEach
void teardown() {
wdm.quit();
}
@Test
void test() {
// Your test logic here
}
}
```
## Support
WebDriverManager is part of [OpenCollective], an online funding platform for open and transparent communities. You can support the project by contributing as a backer (i.e., a personal [donation] or [recurring contribution]) or as a [sponsor] (i.e., a recurring contribution by a company).
### Backers
<a href="https://opencollective.com/webdrivermanager" target="_blank"><img src="https://opencollective.com/webdrivermanager/backers.svg?width=890"></a>
### Sponsors
<a href="https://opencollective.com/webdrivermanager/sponsor/0/website" target="_blank"><img src="https://opencollective.com/webdrivermanager/sponsor/0/avatar.svg"></a>
<a href="https://opencollective.com/webdrivermanager/sponsor/1/website" target="_blank"><img src="https://opencollective.com/webdrivermanager/sponsor/1/avatar.svg"></a>
<a href="https://opencollective.com/webdrivermanager/sponsor/2/website" target="_blank"><img src="https://opencollective.com/webdrivermanager/sponsor/2/avatar.svg"></a>
<a href="https://opencollective.com/webdrivermanager/sponsor/3/website" target="_blank"><img src="https://opencollective.com/webdrivermanager/sponsor/3/avatar.svg"></a>
<a href="https://opencollective.com/webdrivermanager/sponsor/4/website" target="_blank"><img src="https://opencollective.com/webdrivermanager/sponsor/4/avatar.svg"></a>
<a href="https://opencollective.com/webdrivermanager/sponsor/5/website" target="_blank"><img src="https://opencollective.com/webdrivermanager/sponsor/5/avatar.svg"></a>
<a href="https://opencollective.com/webdrivermanager/sponsor/6/website" target="_blank"><img src="https://opencollective.com/webdrivermanager/sponsor/6/avatar.svg"></a>
<a href="https://opencollective.com/webdrivermanager/sponsor/7/website" target="_blank"><img src="https://opencollective.com/webdrivermanager/sponsor/7/avatar.svg"></a>
<a href="https://opencollective.com/webdrivermanager/sponsor/8/website" target="_blank"><img src="https://opencollective.com/webdrivermanager/sponsor/8/avatar.svg"></a>
<a href="https://opencollective.com/webdrivermanager/sponsor/9/website" target="_blank"><img src="https://opencollective.com/webdrivermanager/sponsor/9/avatar.svg"></a>
Alternatively, you can acknowledge my work by buying me a coffee:
<p><a href="https://www.buymeacoffee.com/bonigarcia"> <img align="left" src="https://cdn.buymeacoffee.com/buttons/v2/default-yellow.png" height="50" width="210"/></a></p><br><br>
## About
WebDriverManager (Copyright © 2015-2024) is a project created and maintained by [Boni Garcia] and licensed under the terms of the [Apache 2.0 License].
[Logo]: https://bonigarcia.github.io/img/wdm.png
[WebDriverManager]: https://bonigarcia.dev/webdrivermanager/
[Selenium WebDriver]: https://www.selenium.dev/documentation/webdriver/
[JUnit 5]: https://junit.org/junit5/
[Docker]: https://www.docker.com/
[Docker Engine]: https://docs.docker.com/engine/
[Docker Hub]: https://hub.docker.com/
[noVNC]: https://novnc.com/
[OpenCollective]: https://opencollective.com/webdrivermanager
[donation]: https://opencollective.com/webdrivermanager/donate
[recurring contribution]: https://opencollective.com/webdrivermanager/contribute/backer-8132/checkout
[sponsor]: https://opencollective.com/webdrivermanager/contribute/sponsor-8133/checkout
[Boni Garcia]: https://bonigarcia.dev/
[Apache 2.0 License]: https://www.apache.org/licenses/LICENSE-2.0
| 0 |
macrozheng/mall-tiny | mall-tiny是一款基于SpringBoot+MyBatis-Plus的快速开发脚手架,拥有完整的权限管理功能,可对接Vue前端,开箱即用。 | docker mybatis mybatis-plus mysql redis springboot springsecurity | # mall-tiny
<p>
<a href="#公众号"><img src="http://macro-oss.oss-cn-shenzhen.aliyuncs.com/mall/badge/%E5%85%AC%E4%BC%97%E5%8F%B7-macrozheng-blue.svg" alt="公众号"></a>
<a href="#公众号"><img src="http://macro-oss.oss-cn-shenzhen.aliyuncs.com/mall/badge/%E4%BA%A4%E6%B5%81-%E5%BE%AE%E4%BF%A1%E7%BE%A4-2BA245.svg" alt="交流"></a>
<a href="https://github.com/macrozheng/mall"><img src="http://macro-oss.oss-cn-shenzhen.aliyuncs.com/mall/badge/%E5%90%8E%E5%8F%B0%E9%A1%B9%E7%9B%AE-mall-blue.svg" alt="后台项目"></a>
<a href="https://github.com/macrozheng/mall-admin-web"><img src="http://macro-oss.oss-cn-shenzhen.aliyuncs.com/mall/badge/%E5%89%8D%E7%AB%AF%E9%A1%B9%E7%9B%AE-mall--admin--web-green.svg" alt="前端项目"></a>
</p>
## 友情提示
> 1. **快速体验项目**:[在线访问地址](https://www.macrozheng.com/admin/index.html) 。
> 2. **全套学习教程**:[《mall学习教程》](https://www.macrozheng.com) 。
> 3. **视频教程(2023最新版)**:[《mall视频教程》](https://www.macrozheng.com/mall/catalog/mall_video.html) 。
> 4. **微服务版本**:基于Spring Cloud 2021 & Alibaba的项目:[mall-swarm](https://github.com/macrozheng/mall-swarm) 。
> 5. **SpringBoot 3.x版本**:已支持SpringBoot 3.x + JDK 17,具体参考[3.x分支](https://github.com/macrozheng/mall-tiny/tree/3.x) 。
## 简介
mall-tiny是一款基于SpringBoot+MyBatis-Plus的快速开发脚手架,拥有完整的权限管理功能,可对接Vue前端,开箱即用。
## 项目演示
mall-tiny项目可无缝对接`mall-admin-web`前端项目,秒变权限管理系统。前端项目地址:https://github.com/macrozheng/mall-admin-web

## 技术选型
| 技术 | 版本 | 说明 |
| ---------------------- | ------- | ---------------- |
| SpringBoot | 2.7.0 | 容器+MVC框架 |
| SpringSecurity | 5.7.1 | 认证和授权框架 |
| MyBatis | 3.5.9 | ORM框架 |
| MyBatis-Plus | 3.5.1 | MyBatis增强工具 |
| MyBatis-Plus Generator | 3.5.1 | 数据层代码生成器 |
| Swagger-UI | 3.0.0 | 文档生产工具 |
| Redis | 5.0 | 分布式缓存 |
| Docker | 18.09.0 | 应用容器引擎 |
| Druid | 1.2.9 | 数据库连接池 |
| Hutool | 5.8.0 | Java工具类库 |
| JWT | 0.9.1 | JWT登录支持 |
| Lombok | 1.18.24 | 简化对象封装工具 |
## 数据库表结构

- 化繁为简,仅保留了权限管理功能相关的9张表,方便自由定制;
- 数据库源文件地址:https://github.com/macrozheng/mall-tiny/blob/master/sql/mall_tiny.sql
## 使用流程
### 环境搭建
简化依赖服务,只需安装最常用的MySql和Redis服务即可,服务安装具体参考[mall在Windows环境下的部署](https://www.macrozheng.com/mall/deploy/mall_deploy_windows.html) ,数据库中需要导入`mall_tiny.sql`脚本。
### 开发规约
#### 项目包结构
``` lua
src
├── common -- 用于存放通用代码
| ├── api -- 通用结果集封装类
| ├── config -- 通用配置类
| ├── domain -- 通用封装对象
| ├── exception -- 全局异常处理相关类
| └── service -- 通用业务类
├── config -- SpringBoot中的Java配置
├── domain -- 共用封装对象
├── generator -- MyBatis-Plus代码生成器
├── modules -- 存放业务代码的基础包
| └── ums -- 权限管理模块业务代码
| ├── controller -- 该模块相关接口
| ├── dto -- 该模块数据传输封装对象
| ├── mapper -- 该模块相关Mapper接口
| ├── model -- 该模块相关实体类
| └── service -- 该模块相关业务处理类
└── security -- SpringSecurity认证授权相关代码
├── annotation -- 相关注解
├── aspect -- 相关切面
├── component -- 认证授权相关组件
├── config -- 相关配置
└── util -- 相关工具类
```
#### 资源文件说明
``` lua
resources
├── mapper -- MyBatis中mapper.xml存放位置
├── application.yml -- SpringBoot通用配置文件
├── application-dev.yml -- SpringBoot开发环境配置文件
├── application-prod.yml -- SpringBoot生产环境配置文件
└── generator.properties -- MyBatis-Plus代码生成器配置
```
#### 接口定义规则
- 创建表记录:POST /{控制器路由名称}/create
- 修改表记录:POST /{控制器路由名称}/update/{id}
- 删除指定表记录:POST /{控制器路由名称}/delete/{id}
- 分页查询表记录:GET /{控制器路由名称}/list
- 获取指定记录详情:GET /{控制器路由名称}/{id}
- 具体参数及返回结果定义可以运行代码查看Swagger-UI的Api文档:http://localhost:8080/swagger-ui/

### 项目运行
直接运行启动类`MallTinyApplication`的`main`函数即可。
### 业务代码开发流程
#### 创建业务表
> 创建好`pms`模块的所有表,需要注意的是一定要写好表字段的`注释`,这样实体类和接口文档中就会自动生成字段说明了。

#### 使用代码生成器
> 运行`MyBatisPlusGenerator`类的main方法来生成代码,可直接生成controller、service、mapper、model、mapper.xml的代码,无需手动创建。
- 代码生成器支持两种模式,一种生成单表的代码,比如只生成`pms_brand`表代码可以先输入`pms`,后输入`pms_brand`;

- 生成代码结构一览;

- 另一种直接生成整个模块的代码,比如生成`pms`模块代码可以先输入`pms`,后输入`pms_*`。

#### 编写业务代码
##### 单表查询
> 由于MyBatis-Plus提供的增强功能相当强大,单表查询几乎不用手写SQL,直接使用ServiceImpl和BaseMapper中提供的方法即可。
比如我们的菜单管理业务实现类`UmsMenuServiceImpl`中的方法都直接使用了这些方法。
```java
/**
* 后台菜单管理Service实现类
* Created by macro on 2020/2/2.
*/
@Service
public class UmsMenuServiceImpl extends ServiceImpl<UmsMenuMapper,UmsMenu>implements UmsMenuService {
@Override
public boolean create(UmsMenu umsMenu) {
umsMenu.setCreateTime(new Date());
updateLevel(umsMenu);
return save(umsMenu);
}
@Override
public boolean update(Long id, UmsMenu umsMenu) {
umsMenu.setId(id);
updateLevel(umsMenu);
return updateById(umsMenu);
}
@Override
public Page<UmsMenu> list(Long parentId, Integer pageSize, Integer pageNum) {
Page<UmsMenu> page = new Page<>(pageNum,pageSize);
QueryWrapper<UmsMenu> wrapper = new QueryWrapper<>();
wrapper.lambda().eq(UmsMenu::getParentId,parentId)
.orderByDesc(UmsMenu::getSort);
return page(page,wrapper);
}
@Override
public List<UmsMenuNode> treeList() {
List<UmsMenu> menuList = list();
List<UmsMenuNode> result = menuList.stream()
.filter(menu -> menu.getParentId().equals(0L))
.map(menu -> covertMenuNode(menu, menuList)).collect(Collectors.toList());
return result;
}
@Override
public boolean updateHidden(Long id, Integer hidden) {
UmsMenu umsMenu = new UmsMenu();
umsMenu.setId(id);
umsMenu.setHidden(hidden);
return updateById(umsMenu);
}
}
```
##### 分页查询
> 对于分页查询MyBatis-Plus原生支持,不需要再整合其他插件,直接构造Page对象,然后调用ServiceImpl中的page方法即可。
```java
/**
* 后台菜单管理Service实现类
* Created by macro on 2020/2/2.
*/
@Service
public class UmsMenuServiceImpl extends ServiceImpl<UmsMenuMapper,UmsMenu>implements UmsMenuService {
@Override
public Page<UmsMenu> list(Long parentId, Integer pageSize, Integer pageNum) {
Page<UmsMenu> page = new Page<>(pageNum,pageSize);
QueryWrapper<UmsMenu> wrapper = new QueryWrapper<>();
wrapper.lambda().eq(UmsMenu::getParentId,parentId)
.orderByDesc(UmsMenu::getSort);
return page(page,wrapper);
}
}
```
##### 多表查询
> 对于多表查询,我们需要手写mapper.xml中的SQL实现,由于之前我们已经生成了mapper.xml文件,所以我们直接在Mapper接口中定义好方法,然后在mapper.xml写好SQL实现即可。
- 比如说我们需要写一个根据用户ID获取其分配的菜单的方法,首先我们在`UmsMenuMapper`接口中添加好`getMenuList`方法;
```java
/**
* <p>
* 后台菜单表 Mapper 接口
* </p>
*
* @author macro
* @since 2020-08-21
*/
public interface UmsMenuMapper extends BaseMapper<UmsMenu> {
/**
* 根据后台用户ID获取菜单
*/
List<UmsMenu> getMenuList(@Param("adminId") Long adminId);
}
```
- 然后在`UmsMenuMapper.xml`添加该方法的对应SQL实现即可。
```xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.macro.mall.tiny.modules.ums.mapper.UmsMenuMapper">
<select id="getMenuList" resultType="com.macro.mall.tiny.modules.ums.model.UmsMenu">
SELECT
m.id id,
m.parent_id parentId,
m.create_time createTime,
m.title title,
m.level level,
m.sort sort,
m.name name,
m.icon icon,
m.hidden hidden
FROM
ums_admin_role_relation arr
LEFT JOIN ums_role r ON arr.role_id = r.id
LEFT JOIN ums_role_menu_relation rmr ON r.id = rmr.role_id
LEFT JOIN ums_menu m ON rmr.menu_id = m.id
WHERE
arr.admin_id = #{adminId}
AND m.id IS NOT NULL
GROUP BY
m.id
</select>
</mapper>
```
### 项目部署
mall-tiny已经集成了Docker插件,可以打包成Docker镜像来部署,具体参考:[使用Maven插件为SpringBoot应用构建Docker镜像](https://www.macrozheng.com/project/maven_docker_fabric8.html)
安装好MySQL和Redis服务后,直接使用如下命令运行即可。
```bash
docker run -p 8080:8080 --name mall-tiny \
--link mysql:db \
--link redis:redis \
-e 'spring.profiles.active'=prod \
-v /etc/localtime:/etc/localtime \
-v /mydata/app/mall-tiny/logs:/var/logs \
-d mall-tiny/mall-tiny:1.0.0-SNAPSHOT
```
### 其他说明
#### SpringSecurity相关
> 由于使用了SpringSecurity来实现认证和授权,部分接口需要token才可以访问,访问需要认证授权接口流程如下。
- 访问Swagger-UI接口文档:http://localhost:8080/swagger-ui/
- 调用登录接口获取token;

- 点击右上角Authorize按钮输入token,然后访问相关接口即可。

#### 请求参数校验
> 默认集成了`Jakarta Bean Validation`参数校验框架,只需在参数对象属性中添加`javax.validation.constraints`包中的注解注解即可实现校验功能,这里以登录参数校验为例。
- 首先在登录请求参数中添加`@NotEmpty`注解;
```java
/**
* 用户登录参数
* Created by macro on 2018/4/26.
*/
@Data
@EqualsAndHashCode(callSuper = false)
public class UmsAdminLoginParam {
@NotEmpty
@ApiModelProperty(value = "用户名",required = true)
private String username;
@NotEmpty
@ApiModelProperty(value = "密码",required = true)
private String password;
}
```
- 然后在登录接口中添加`@Validated`注解开启参数校验功能即可。
```java
/**
* 后台用户管理
* Created by macro on 2018/4/26.
*/
@Controller
@Api(tags = "UmsAdminController", description = "后台用户管理")
@RequestMapping("/admin")
public class UmsAdminController {
@ApiOperation(value = "登录以后返回token")
@RequestMapping(value = "/login", method = RequestMethod.POST)
@ResponseBody
public CommonResult login(@Validated @RequestBody UmsAdminLoginParam umsAdminLoginParam) {
String token = adminService.login(umsAdminLoginParam.getUsername(), umsAdminLoginParam.getPassword());
if (token == null) {
return CommonResult.validateFailed("用户名或密码错误");
}
Map<String, String> tokenMap = new HashMap<>();
tokenMap.put("token", token);
tokenMap.put("tokenHead", tokenHead);
return CommonResult.success(tokenMap);
}
}
```
## 公众号
加微信群交流,关注公众号「**macrozheng**」回复「**加群**」即可。
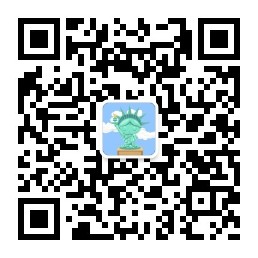
## 许可证
[Apache License 2.0](https://github.com/macrozheng/mall-tiny/blob/master/LICENSE)
Copyright (c) 2018-2022 macrozheng
| 0 |
spring2go/staffjoy | 微服务(Microservices)和云原生架构教学案例项目,基于Spring Boot和Kubernetes技术栈 | cloud-native kubernetes microservice spring-boot | # Staffjoy 教学版
微服务和云原生架构教学案例项目,基于 Spring Boot 和 Kubernetes 技术栈
<img src="doc/images/bobo_promote.jpg" width="500" height="400">
## 课程资料 PPT
1. 第 1 章 [课程介绍和案例需求](https://github.com/spring2go/staffjoy-ppt/blob/master/doc/ppts/Chapter_01.pdf)
2. 第 2 章 [系统架构设计和技术栈选型](https://github.com/spring2go/staffjoy-ppt/blob/master/doc/ppts/Chapter_02.pdf)
3. 第 3 章 [服务开发框架设计和实践](https://github.com/spring2go/staffjoy-ppt/blob/master/doc/ppts/Chapter_03.pdf)
4. 第 4 章 [可编程网关设计和实践](https://github.com/spring2go/staffjoy-ppt/blob/master/doc/ppts/Chapter_04.pdf)
5. 第 5 章 [安全框架设计和实践](https://github.com/spring2go/staffjoy-ppt/blob/master/doc/ppts/Chapter_05.pdf)
6. 第 6 章 [微服务测试设计和实践](https://github.com/spring2go/staffjoy-ppt/blob/master/doc/ppts/Chapter_06.pdf)
7. 第 7 章 [可运维架构设计和实践](https://github.com/spring2go/staffjoy-ppt/blob/master/doc/ppts/Chapter_07.pdf)
8. 第 8 章 [服务容器化和 Docker Compose 部署](https://github.com/spring2go/staffjoy-ppt/blob/master/doc/ppts/Chapter_08.pdf)
9. 第 9 章 [云原生架构和 Kubernetes 容器云部署](https://github.com/spring2go/staffjoy-ppt/blob/master/doc/ppts/Chapter_09.pdf)
10. 第 10 章 [项目复盘、扩展和应用](https://github.com/spring2go/staffjoy-ppt/blob/master/doc/ppts/Chapter_10.pdf)
11. 第 11 章 [附录:Staffjoy 微服务实现简析](https://github.com/spring2go/staffjoy-ppt/blob/master/doc/ppts/Chapter_11.pdf)
12. 附:[课程参考资料链接](doc/reference.md)
## 项目初衷
微服务和云原生架构是目前互联网行业的技术热点,相关资料文档很多,但是缺乏端到端的贴近生产的案例,这就使得很多互联网开发人员(包括架构师),虽然学习了很多微服务理论,但是在真正落地实施微服务云原生架构的时候,仍然会感到困惑。为此,我利用业余时间,通过改造一个叫[Staffjoy](https://github.com/staffjoy/v2)的开源项目,开发了这个教学版的案例项目。整个项目采用微服务架构,并且可以一键部署到 Kubernetes 容器云环境。最近我和极客时间合作,基于这个案例项目开发了一门课程《Spring Boot 与 Kubernetes 云原生微服务实践 ~ 全面掌握云原生应用的架构设计与实现》,参考[课程大纲](doc/syllabus.md)。希望通过实际案例项目和课程的学习,让开发人员/架构师不仅能够深入理解微服务和云原生架构原理,同时能够在生产实践中真正地去落地实施微服务和云原生架构。也希望这个项目成为微服务云原生架构的一个参考模版,进一步可以作为类似项目的脚手架。
## 课程目标
通过具体案例的形式,教大家使用 SpringBoot 框架,开发一个贴近生产的微服务应用,并一键部署到 Kubernetes 容器云环境,帮助大家:
1. 掌握如何在实践中设计微服务架构和前后分离架构
2. 掌握如何基于 SpringBoot 搭建微服务基础框架
3. 掌握 SpringBoot 测试技术和相关实践
4. 掌握服务容器化和容器云部署相关实践
5. 进一步提升 Java/Spring 微服务开发技能
6. 理解可运维架构理念和相关实践
7. 理解如何架构和设计一个 SaaS 多租户应用
8. 理解云时代的 DevOps 工程实践
## 项目架构

- **Account API(账户服务)**,提供账户注册、登录认证和账户信息管理等基本功能。
- **Company API(公司服务)**,支持团队(Team),雇员(Worker),任务(Job)和班次(Shift)等核心领域概念的管理功能。
- **Bot API**,是一个消息转发服务,它一方面作为队列可以缓冲高峰期的大量通知消息,另一方面作为代理可以屏蔽将来可能的通知方式的变更。
- **Mail Sender 和 SMS Sender**,都是消息通知服务,分别支持邮件和短信通知方式,它们可以对接各种云服务,比如阿里云邮件或短信服务。
- **WhoAmI API**,支持前端应用获取当前登录用户的详情信息,包括公司和管理员身份,团队信息等,它也可以看作是一个用户会话(Session)信息服务。
- **App(也称 MyCompany)**,单页 SPA 应用,是整个 Staffjoy 应用的主界面,公司管理员通过它管理公司、雇员、任务和排班等信息。
- **MyAccount** ,单页 SPA 应用,它主要支持公司雇员管理个人信息,包括邮件和电话等,方便接收排班通知消息。
- **WWW 应用**, 是一个前端 MVC 应用,它主要支持产品营销、公司介绍和用户注册登录/登出,这个应用也称为营销站点(Marketing Site)或者登录页(Landing Page)应用。
- **Faraday(法拉弟)**,是一个反向代理(功能类似 nginx),也可以看作是一个网关(功能类似 zuul),它是用户访问 Staffjoy 微服务应用的流量入口,它既实现对前端应用和后端 API 的路由访问,也实现登录鉴权和访问控制等安全功能。Faraday 代理是 Staffjoy 微服务架构和前后分离架构的关键,并且它是唯一具有公网 IP 的服务。
Staffjoy 微服务间通讯,包括对外暴露 API,全部采用 JSON over HTTP 标准方式。

上图是经过调用链埋点监控后,在 Skywalking Dashboard 上实时呈现出来的服务依赖关系图,这个依赖图和总体架构设计保持一致。
## 项目技术栈
- Spring Boot
- Spring REST
- Spring Data JPA
- Spring MVC + Thymeleaf
- MySql
- ReactJs + Redux
- Docker Compose
- Kubernetes
Staffjoy 教学版所采用的技术栈都是目前行业主流,数量不多,如上面架构图所标示。所有微服务(绿色标注)采用**Spring REST**开发,有数据访问交互的采用**Spring Data JPA**,数据库使用**MySQL**。WWW 服务使用**Spring MVC+Thymeleaf**模版引擎开发。Faraday 也是一个**SpringBoot**应用,内部路由和安全等逻辑基于**Servlet Filter**实现。两个单页 SPA 应用(暗红色标注)都是采用**ReactJs+Redux**框架开发。整个应用支持一键部署到本地**Docker Compose**环境,也支持一键部署到**Kubernetes**容器云环境,所以 Staffjoy 的整体架构是支持云原生的微服务架构。
## 关于项目的进一步说明
1. 教学版 Staffjoy 和原版 Staffjoy 在功能、设计和实现逻辑上基本保持一致,但教学版在原版基础上做了一些改造,以适应教学需要。首先,在开发语言框架上,原版 Staffjoy 用 Golang/Grpc 实现微服务,教学版 Staffjoy 则改造为用国内更主流的 Spring(Boot)实现微服务;其次,在架构上,原版 Staffjoy 因为使用 Grpc 开发微服务,为了将 Rpc 服务暴露成 HTTP/REST 服务,它多一个对应的 Grpc API Gateway 转换层服务,而教学版 Staffjoy 因为使用 Spring(Boot)开发,直接支持 HTTP/REST 接口,所以不需要独立转换层服务;第三,原版 Staffjoy 默认使用 SMS 短信发送排班通知信息,但在国内开通短信服务需要审批,比较麻烦,所以在教学版 Staffjoy 中,排班通知默认调整为邮件方式,方便测试和演示。学习理解了 Spring(Boot)教学版 Staffjoy,很容易理解原版 Golang/Grpc 开发的 Staffjoy,对原版有兴趣的学员可以直接看官方[源码](https://github.com/Staffjoy/v2)。
2. 开发和运行教学版 Staffjoy,需要安装一些必要的开发工具(操作系统不限),包括 JDK8,Maven 依赖管理工具,Intellij IDEA 或者 Eclipse STS IDE,MySQL 数据库和 MySQL Workbench 管理工具,Nodejs/npm 前端开发框架,Postman API 测试工具,以及 Docker 运行时环境。因为 Staffjoy 服务较多,如果要在本机跑,建议物理内存**不少于 8G**。
3. 教学版的 Staffjoy 虽然是一个较完整的 SaaS 应用,并且架构设计中考虑了很多生产性环节,但是它仍然只是一个教学演示项目,仅供学习参考,如果你要将它进行生产化应用(或者基于它的代码做其它项目的脚手架),则仍然需要对其进行严格测试和定制扩展。大家在学习或使用教学版 Staffjoy 过程中,如果发现有 bug,或者对项目有完善扩展建议,欢迎提交 github issue.
## 如何运行
1. 配置文件
Staffjoy 教学版依赖一些私密配置,例如 sentry-dsn 和 aliyun-access-key 等等,这些私密配置不能 checkin 到 github 上,所以采用了 Spring 的一种私密配置机制,私密数据集中配置在**config/application.yml**中,这个文件在 gitignore 中,不会被 checkin 到 github。请参考 config 目录中的[application.yml.example](config/application.yml.example)文件和格式,在 config 目录中添加一个**appliction.yml**文件,其中填写你自己的私密配置。如果你暂时没有这些配置,可以暂时用假数据,直接把 application.yml.example,改为 application.yml,这样应用可以运行起来。注意,如果 aliyun 相关配置不配,则无法发送邮件或短信,sentry 相关配置不配则无法发送异常数据到 sentry,intercom 不配则不能对接 intercom 客服系统,recaptcha 暂未用可以不配。
**关于如何运行的进一步内容,请参考极客时间的视频课程,课程里头有step by step演示**。
### 注意!!!
Staffjoy 的 mail-svc 依赖于阿里云的邮件推送(DirectMail)发送邮件,不少学员反应运行时邮件发送不成功,实际配置阿里云 DirectMail 是比较麻烦的,有一个简单办法可以绕开:邮件发送不成功的话,会报异常日志,日志里头有邮件内容,比如账号注册邮件发不成功,可以从异常日志里头找到激活链接,把链接贴到浏览器中,就可以激活账号了。后续考虑简化 mail-svc,支持 smtp 等简单方式配置和发送邮件。
## Staffjoy 公司和案例背景
[Staffjoy](https://www.staffjoy.com/)曾经是美国硅谷的一家初创公司,成立于 2015 年,创始人是[Philip I. Thomas](https://www.linkedin.com/in/philipithomas/),公司曾获得 Y Combinator 等知名机构的投资。Staffjoy 的主要业务是为小企业提供工时排班(Scheduling)软件解决方案,帮助企业提升雇员管理效率,主要面向零售、餐饮等服务行业。因业务发展和招聘等原因,[Staffjoy 公司最终于 2017 年关闭](https://blog.staffjoy.com/denouement-abe7d26f2de0),在关闭前,公司把核心产品大部分都[开源](https://github.com/Staffjoy)贡献给了 Github 社区。[Staffjoy V2](https://github.com/Staffjoy/v2)是公司关闭前研发的最新一款 SaaS 版企业排班系统,目前在 Github 上有超过 1k 星,总体设计和代码质量较高,目前有不少企业在定制使用。Staffjoy V2 是一个小规模 SaaS 应用,采用微服务和前后分离架构,支持 Kubernetes/GKE 容器云环境一键部署,是学习现代 SaaS、微服务和云原生架构的一个模版项目。
## Staffjoy 应用的功能需求
Staffjoy 应用的业务功能相对简单,简单讲就是帮助小企业管理者管理雇员和排班,并以短信或者邮件等方式,将排班信息及时通知到雇员。具体讲,Staffjoy 主要支持两类用户角色和用例,一类是公司管理员(admin),他们可以通过 Staffjoy 管理公司(company)、员工目录(directory),团队(team)和雇员(worker),也可以创建任务(job),创建和发布班次(shift)信息;另一类是公司雇员,他们可以通过 Staffjoy 管理电话和邮件等个人信息,便于接收到对应的排班通知。Staffjoy 应用主要以共享版 SaaS 服务形式提供,也支持针对一些大客户的定制私有部署,这就要求 Staffjoy 应用易于部署和运维,要支持一键部署到 GKE 等容器云环境。另外,作为一款 SaaS 服务产品,良好的市场营销(Marketing)和客服是赢得用户的关键,所以 Staffjoy 需要提供营销友好的(Marketing Friendly)宣传和登录页(Landing Page),也要支持对接主流的在线客服系统如 Intercom。
## 项目界面预览
### 1. 首页

### 2. 订购计划和价格页

### 3. 登录页

### 4. 雇员账户管理 SPA 单页应用

### 5. 我的公司 SPA 单页应用

## 其它可供参考微服务案例项目
- [eShopOnContainers](https://github.com/dotnet-architecture/eShopOnContainers) 微软支持
- [microservices-demo](https://github.com/GoogleCloudPlatform/microservices-demo) 谷歌支持
- [piggy-metrics](https://github.com/sqshq/piggymetrics)
| 0 |
piomin/sample-spring-microservices-new | Demo for Spring Boot 3(`master` branch)/2(other branches) and Spring Cloud microservices with distributed configuration (Spring Cloud Config), service discovery (Eureka), API gateway (Spring Cloud Gateway, Zuul), Swagger/OpenAPI documentation (Springdoc), logs correlation using Spring Cloud Sleuth/Micrometer OTEL and many more | eureka feign microservices netty ribbon service-discovery spring-boot spring-boot-2 spring-boot-3 spring-cloud spring-cloud-config spring-cloud-gateway spring-cloud-netflix spring-cloud-sleuth swagger2 zuul | null | 0 |
in28minutes/master-spring-and-spring-boot | Spring and Spring Boot Tutorial For Absolute Beginners - 10-in-1 - Spring to Spring Boot to REST API to Full Stack to Containers to Cloud | aws docker java spring spring-boot spring-security | # Master Spring and Spring Boot
**Spring and Spring Boot** Frameworks are the **No 1 frameworks** for building enterprise apps in the Java world.
In this course, you will **learn Spring and Spring Boot from ZERO**.
I'm a great believer that the best way to learn is by doing and we designed this course to be **hands-on**.
You will build a **web application, a REST API and full stack application** using Spring, Spring Boot, JPA, Hibernate, React, Spring Security, Maven and Gradle.
You will learn to containerise applications using Docker. You will learn to deploy these applications to AWS.
By the end of the course, you will know everything you would need to become a great Spring and Spring Boot Developer.
## Installing Tools
### Our Recommendations
- Use **latest version** of Java
- Use **latest version** of "Eclipse IDE for Enterprise Java Developers"
- Remember: Spring Boot 3+ works only with Java 17+
### Installing Java
- Windows - https://www.youtube.com/watch?v=I0SBRWVS0ok
- Linux - https://www.youtube.com/watch?v=mHvFpyHK97A
- Mac - https://www.youtube.com/watch?v=U3kTdMPlgsY
#### Troubleshooting
- Troubleshooting Java Installation - https://www.youtube.com/watch?v=UI_PabQ1YB0
### Installing Eclipse
- Windows - https://www.youtube.com/watch?v=toY06tsME-M
- Others - https://www.youtube.com/watch?v=XveQ9Gq41UM
#### Troubleshooting
- Configuring Java in Eclipse - https://www.youtube.com/watch?v=8i0r_fcE3L0
## Lectures
### Getting Started - Master Spring Framework and Spring Boot
- Getting Started - Master Spring Framework and Spring Boot
### Getting Started with Java Spring Framework
- Step 01 - Understanding the Need for Java Spring Framework
- Step 02 - Getting Started with Java Spring Framework
- Step 03 - Creating a New Spring Framework Project with Maven and Java
- Step 04 - Getting Started with Java Gaming Application
- Step 05 - Understanding Loose Coupling and Tight Coupling
- Step 06 - Introducting Java Interface to Make App Loosely Coupled
- Step 07 - Bringing in Spring Framework to Make Java App Loosely Coupled
- Step 08 - Your First Java Spring Bean and Launching Java Spring Configuration
- Step 09 - Creating More Java Spring Beans in Spring Java Configuration File
- Step 10 - Implementing Auto Wiring in Spring Framework Java Configuration File
- Step 11 - Questions about Spring Framework - What will we learn?
- Step 12 - Understanding Spring IOC Container - Application Context and Bean Factory
- Step 13 - Exploring Java Bean vs POJO vs Spring Bean
- Step 14 - Exploring Spring Framework Bean Auto Wiring - Primary and Qualifier Annotations
- Step 15 - Using Spring Framework to Manage Beans for Java Gaming App
- Step 16 - More Questions about Java Spring Framework - What will we learn?
- Step 17 - Exploring Spring Framework With Java - Section 1 - Review
### Using Spring Framework to Create and Manage Your Java Objects
- Step 01 - Getting Spring Framework to Create and Manage Your Java Objects
- Step 02 - Exploring Primary and Qualifier Annotations for Spring Components
- Step 03 - Primary and Qualifier - Which Spring Annotation Should You Use?
- Step 04 - Exploring Spring Framework - Different Types of Dependency Injection
- Step 05 - Java Spring Framework - Understanding Important Terminology
- Step 06 - Java Spring Framework - Comparing @Component vs @Bean
- Step 07 - Why do we have dependencies in Java Spring Applications?
- Step 08 - Exercise/ Solution for Real World Java Spring Framework Example
- Step 09 - Exploring Spring Framework With Java - Section 2 - Review
### Exploring Spring Framework Advanced Features
- Step 01 - Exploring Lazy and Eager Initialization of Spring Framework Beans
- Step 02 - Comparing Lazy Initialization vs Eager Initialization
- Step 03 - Exploring Java Spring Framework Bean Scopes - Prototype and Singleton
- Step 04 - Comparing Prototype vs Singleton - Spring Framework Bean Scopes
- Step 05 - Exploring Spring Beans - PostConstruct and PreDestroy
- Step 06 - Evolution of Jakarta EE - Comparing with J2EE and Java EE
- Step 07 - Exploring Jakarta CDI with Spring Framework and Java
- Step 08 - Exploring Java Spring XML Configuration
- Step 09 - Exploring Java Annotations vs XML Configuration for Java Spring Framework
- Step 10 - Exploring Spring Framework Stereotype Annotations - Component and more
- Step 11 - Quick Review - Important Spring Framework Annotations
- Step 12 - Quick Review - Important Spring Framework Concepts
- Step 13 - Exploring Spring Big Picture - Framework, Modules and Projects
### Getting Started with Spring Boot
- Step 01 - Getting Started with Spring Boot - Goals
- Step 02 - Understanding the World Before Spring Boot - 10000 Feet Overview
- Step 03 - Setting up New Spring Boot Project with Spring Initializr
- Step 04 - Build a Hello World API with Spring Boot
- Step 05 - Understanding the Goal of Spring Boot
- Step 06 - Understanding Spring Boot Magic - Spring Boot Starter Projects
- Step 07 - Understanding Spring Boot Magic - Auto Configuration
- Step 08 - Build Faster with Spring Boot DevTools
- Step 09 - Get Production Ready with Spring Boot - 1 - Profiles
- Step 10 - Get Production Ready with Spring Boot - 2 - ConfigurationProperties
- Step 11 - Get Production Ready with Spring Boot - 3 - Embedded Servers
- Step 12 - Get Production Ready with Spring Boot - 4 - Actuator
- Step 13 - Understanding Spring Boot vs Spring vs Spring MVC
- Step 14 - Getting Started with Spring Boot - Review
### Getting Started with JPA and Hibernate with Spring and Spring Boot
- Step 01 - Getting Started with JPA and Hibernate - Goals
- Step 02 - Setting up New Spring Boot Project for JPA and Hibernate
- Step 03 - Launching up H2 Console and Creating Course Table in H2
- Step 04 - Getting Started with Spring JDBC
- Step 05 - Inserting Hardcoded Data using Spring JDBC
- Step 06 - Inserting and Deleting Data using Spring JDBC
- Step 07 - Querying Data using Spring JDBC
- Step 08 - Getting Started with JPA and EntityManager
- Step 09 - Exploring the Magic of JPA
- Step 10 - Getting Started with Spring Data JPA
- Step 11 - Exploring features of Spring Data JPA
- Step 12 - Understanding difference between Hibernate and JPA
### Build Java Web Application with Spring Framework, Spring Boot and Hibernate
- Step 00 - Introduction to Building Web App with Spring Boot
- Step 01 - Creating Spring Boot Web Application with Spring Initializr
- Step 02 - Quick overview of Spring Boot Project
- Step 03 - First Spring MVC Controller, @ResponseBody, @Controller
- Step 04 - 01 - Enhancing Spring MVC Controller to provide HTML response
- Step 04 - 02 - Exploring Step By Step Coding and Debugging Guide
- Step 05 - Redirect to a JSP using Spring Boot - Controller, @ResponseBody and View Resolver
- Step 06 - Exercise - Creating LoginController and login view
- Step 07 - Quick Overview - How does web work - Request and Response
- Step 08 - Capturing QueryParams using RequestParam and First Look at Model
- Step 09 - Quick Overview - Importance of Logging with Spring Boot
- Step 10 - Understanding DispatcherServlet, Model 1, Model 2 and Front Controller
- Step 11 - Creating a Login Form
- Step 12 - Displaying Login Credentials in a JSP using Model
- Step 13 - Add hard coded validation of userid and password
- Step 14 - Getting started with Todo Features - Creating Todo and TodoService
- Step 15 - Creating first version of List Todos Page
- Step 16 - Understanding Session vs Model vs Request - @SessionAttributes
- Step 17 - Adding JSTL to Spring Boot Project and Showing Todos in a Table
- Step 18 - Adding Bootstrap CSS framework to Spring Boot Project using webjars
- Step 19 - Formatting JSP pages with Bootstrap CSS framework
- Step 20 - Lets Add a New Todo - Create a new View
- Step 21 - Enhancing TodoService to add the todo
- Step 22 - Adding Validations using Spring Boot Starter Validation
- Step 23 - Using Command Beans to implement New Todo Page Validations
- Step 24 - Implementing Delete Todo Feature - New View
- Step 25 - Implementing Update Todo - 1 - Show Update Todo Page
- Step 26 - Implementing Update Todo - 1 - Save changes to Todo
- Step 27 - Adding Target Date Field to Todo Page
- Step 28 - Adding a Navigation Bar and Implementing JSP Fragments
- Step 29 - Preparing for Spring Security
- Step 30 - Setting up Spring Security with Spring Boot Starter Security
- Step 31 - Configuring Spring Security with Custom User and Password Encoder
- Step 32 - Refactoring and Removing Hardcoding of User Id
- Step 33 - Setting up a New User for Todo Application
- Step 34 - Adding Spring Boot Starter Data JPA and Getting H2 database ready
- Step 35 - 01 - Configuring Spring Security to Get H2 console Working
- Step 36 - Making Todo an Entity and Population Todo Data into H2
- Step 37 - Creating TodoRepository and Connecting List Todos page from H2 database
- Step 38 - 01 - Connecting All Todo App Features to H2 Database
- Step 38 - 02 - Exploring Magic of Spring Boot Starter JPA and JpaRepository
- Step 39 - OPTIONAL - Overview of Connecting Todo App to MySQL database
- Step 40 - OPTIONAL - Installing Docker
- Step 41 - OPTIONAL - Connecting Todo App to MySQL database
### Creating a Java REST API with Spring Boot, Spring Framework and Hibernate
- Step 00 - Creating a REST API with Spring Boot - An Overview
- Step 01 - Initializing a REST API Project with Spring Boot
- Step 02 - Creating a Hello World REST API with Spring Boot
- Step 03 - Enhancing the Hello World REST API to return a Bean
- Step 04 - What's happening in the background? Spring Boot Starters and Autoconfiguration
- Step 05 - Enhancing the Hello World REST API with a Path Variable
- Step 06 - Designing the REST API for Social Media Application
- Step 07 - Creating User Bean and UserDaoService
- Step 08 - Implementing GET Methods for User Resource
- Step 09 - Implementing POST Method to create User Resource
- Step 10 - Enhancing POST Method to return correct HTTP Status Code and Location URI
- Step 11 - Implementing Exception Handling - 404 Resource Not Found
- Step 12 - Implementing Generic Exception Handling for all Resources
- Step 13 - Implementing DELETE Method to delete a User Resource
- Step 14 - Implementing Validations for REST API
- Step 15 - Overview of Advanced REST API Features
- Step 16 - Understanding Open API Specification and Swagger
- Step 17 - Configuring Auto Generation of Swagger Documentation
- Step 18 - Exploring Content Negotiation - Implementing Support for XML
- Step 19 - Exploring Internationalization for REST API
- Step 20 - Versioning REST API - URI Versioning
- Step 21 - Versioning REST API - Request Param, Header and Content Negotiation
- Step 22 - Implementing HATEOAS for REST API
- Step 23 - Implementing Static Filtering for REST API
- Step 24 - Implementing Dynamic Filtering for REST API
- Step 25 - Monitoring APIs with Spring Boot Actuator
- Step 26 - Exploring APIs with Spring Boot HAL Explorer
- Step 27 - Connecting REST API to H2 using JPA and Hibernate - An Overview
- Step 28 - Creating User Entity and some test data
- Step 29 - Enhancing REST API to connect to H2 using JPA and Hibernate
- Step 30 - Creating Post Entity with Many to One Relationship with User Entity
- Step 31 - Implementing a GET API to retrieve all Posts of a User
- Step 32 - Implementing a POST API to create a Post for a User
- Step 33 - Exploring JPA and Hibernate Queries for REST API
- Step 34 - Connecting REST API to MySQL Database - An Overview
- Step 34z - OPTIONAL - Installing Docker
- Step 35 - OPTIONAL - Connecting REST API to MySQL Database - Implementation
- Step 36 - Implementing Basic Authentication with Spring Security
- Step 37 - Enhancing Spring Security Configuration for Basic Authentication
### Building Java Full Stack Application with Spring Boot and React
- Step 01 - Getting Started - Full Stack Spring Boot and React Application
- Step 02 - Exploring What and Why of Full Stack Architectures
- Step 03 - Understanding JavaScript and EcmaScript History
- Step 04 - Installing Visual Studio Code
- Step 05 - Installing nodejs and npm
- Step 06 - Creating React App with Create React App
- Step 07 - Exploring Important nodejs Commands - Create React App
- Step 08 - Exploring Visual Studio Code and Create React App
- Step 09 - Exploring Create React App Folder Structure
- Step 10 - Getting started with React Components
- Step 11 - Creating Your First React Component and more
- Step 12 - Getting Started with State in React - useState hook
- Step 13 - Exploring JSX - React Views
- Step 14 - Following JavaScript Best Practices - Refactoring to Modules
- Step 15 - Exploring JavaScript further
### Exploring React Components with Counter Example
- Step 01 - Exploring React Components with Counter Example
- Step 02 - Getting Started with React Application - Counter
- Step 03 - Getting Started with React Application - Counter - 2
- Step 04 - Exploring React State with useState hook - Adding state to Counter
- Step 05 - Exploring React State - What is happening in Background?
- Step 06 - Exploring React Props - Setting Counter increment value
- Step 07 - Creating Multiple Counter Buttons
- Step 08 - Moving React State Up - Setting up Counter and Counter Button
- Step 09 - Moving React State Up - Calling Parent Component Methods
- Step 10 - Exploring React Developer Tools
- Step 11 - Adding Reset Button to Counter
- Step 12 - Refactoring React Counter Component
### Building Java Todo Full Stack Application with Spring Boot and React
- Step 01 - Getting Started with React Todo Management App
- Step 02 - Getting Started with Login Component - Todo React App
- Step 03 - Improving Login Component Further - Todo React App
- Step 04 - Adding Hardcoded Authentication - Todo React App
- Step 05 - Conditionally Displaying Messages in Login Component - Todo React App
- Step 06 - Adding React Router Dom and Routing from Login to Welcome Component
- Step 07 - Adding Error Component to our React App
- Step 08 - Removing Hard Coding from Welcome Component
- Step 09 - Getting Started with React List Todo Component
- Step 10 - Displaying More Todo Details in React List Todo Component
- Step 11 - Creating React Header, Footer and Logout Components
- Step 12 - Adding Bootstrap to React Front End Application
- Step 13 - Using Bootstrap to Style Todo React Front End Application
- Step 14 - Refactoring React Components to Individual JavaScript Modules
- Step 15 - Sharing React State with Multiple Components with Auth Context
- Step 16 - Updating React State and Verifying Updates through Auth Context
- Step 17 - Setting isAuthenticated into React State - Auth Context
- Step 18 - Protecting Secure React Routes using Authenticated Route - 1
- Step 19 - Protecting Secure React Routes using Authenticated Route - 2
### Connecting Spring Boot REST API with React Frontend - Java Full Stack Application
- Step 01 - Setting Todo REST API Project for React Full Stack Application
- Step 02 - Calling Spring Boot Hello World REST API from React Hello World Component
- Step 03 - Enabling CORS Requests for Spring Boot REST API
- Step 04 - Invoking Spring Boot Hello World Bean and Path Param REST API from React
- Step 05 - Refactoring Spring Boot REST API Invocation Code to New Module
- Step 06 - Following Axios Best Practices in Spring Boot REST API
- Step 07 - Creating Retrieve Todos Spring Boot REST API Get Method
- Step 08 - Displaying Todos from Spring Boot REST API in React App
- Step 09 - Creating Retrieve Todo and Delete Todo Spring Boot REST API Methods
- Step 10 - Adding Delete Feature to React Frontend
- Step 11 - Setting Username into React Auth Context
- Step 12 - Creating Todo React Component to display Todo Page
- Step 13 - Adding Formik and Moment Libraries to Display Todo React Component
- Step 14 - Adding Validation to Todo React Component using Formik
- Step 15 - Adding Update Todo and Create Todo REST API to Spring Boot Backend API
- Step 16 - Adding Update Feature to React Frontend
- Step 17 - Adding Create New Todo Feature to React Frontend
- Step 18 - Securing Spring Boot REST API with Spring Security
- Step 19 - Adding Authorization Header in React to Spring Boot REST API calls
- Step 20 - Configuring Spring Security to allow all Options Requests
- Step 21 - Calling Basic Authentication Service when Logging into React App
- Step 22 - Using async and await to invoke Basic Auth API
- Step 23 - Setting Basic Auth Token into Auth Context
- Step 24 - Setting up Axios Interceptor to add Authorization Header
- Step 24A - Debugging Problems with Basic Auth and Spring Boot
- Step 25 - Getting Started with JWT and Spring Security
- Step 26 - Integrating Spring Security JWT REST API with React Frontend
- Step 27 - Debugging Problems with JWT Auth and Spring Boot
### Connecting Java Full Stack Application (Spring Boot and React) with JPA and Hibernate
- Step 01 - Full Stack React and Spring Boot with JPA and Hibernate
- Step 02 - Full Stack React & Spring Boot with JPA & Hibernate - Getting Tables Ready
- Step 03 - Full Stack React & Spring Boot with JPA & Hibernate - Todo CRUD operations
- Step 04 - Full Stack React & Spring Boot with JPA & Hibernate - Add New Todo
- Step 05 - Full Stack React & Spring Boot with JPA & Hibernate - Connect with MySQL
### Exploring Unit Testing with JUnit
- Step 01 - What is JUnit and Unit Testing_
- Step 02 - Your First JUnit Project and Green Bar
- Step 03 - Your First Code and First Unit Test
- Step 04 - Exploring other assert methods
- Step 05 - Exploring few important JUnit annotations
### Exploring Mocking with Mockito for Spring Boot Projects
- Step 00 - Introduction to Section - Mockito in 5 Steps
- Step 01 - Setting up a Spring Boot Project
- Step 02 - Understanding problems with Stubs
- Step 03 - Writing your first Mockito test with Mocks
- Step 04 - Simplifying Tests with Mockito Annotations - @Mock, @InjectMocks
- Step 05 - Exploring Mocks further by Mocking List interface
### Securing Spring Boot Applications with Spring Security
- Step 00 - Getting started with Spring Security
- Step 01 - Understanding Security Fundamentals
- Step 02 - Understanding Security Principles
- Step 03 - Getting Started with Spring Security
- Step 04 - Exploring Default Spring Security Configuration
- Step 05 - Creating Spring Boot Project for Spring Security
- Step 06 - Exploring Spring Security - Form Authentication
- Step 07 - Exploring Spring Security - Basic Authentication
- Step 08 - Exploring Spring Security - Cross Site Request Forgery - CSRF
- Step 09 - Exploring Spring Security - CSRF for REST API
- Step 10 - Creating Spring Security Configuration to Disable CSRF
- Step 11 - Exploring Spring Security - Getting Started with CORS
- Step 12 - Exploring Spring Security - Storing User Credentials in memory
- Step 13 - Exploring Spring Security - Storing User Credentials using JDBC
- Step 14 - Understanding Encoding vs Hashing vs Encryption
- Step 15 - Exploring Spring Security - Storing Bcrypt Encoded Passwords
- Step 16 - Getting Started with JWT Authentication
- Step 17 - Setting up JWT Auth with Spring Security and Spring Boot - 1
- Step 18 - Setting up JWT Auth with Spring Security and Spring Boot - 2
- Step 19 - Setting up JWT Resource with Spring Security and Spring Boot - 1
- Step 20 - Setting up JWT Resource with Spring Security and Spring Boot - 2
- Step 21 - Understanding Spring Security Authentication
- Step 22 - Exploring Spring Security Authorization
- Step 23 - Creating a Spring Boot Project for OAuth with Spring Security
- Step 24 - Getting Started with Spring Boot and OAuth2 - Login with Google
- Step 25 - Quick Review - Securing Spring Boot Apps with Spring Security
### Learning Spring AOP with Spring Boot
- Step 01 - Getting Started with Spring AOP - An overview
- Step 02 - What is Aspect Oriented Programming?
- Step 03 - Creating a Spring Boot Project for Spring AOP
- Step 04 - Setting up Spring Components for Spring AOP
- Step 05 - Creating AOP Logging Aspect and Pointcut
- Step 06 - Understanding AOP Terminology
- Step 07 - Exploring @After, @AfterReturning, @AfterThrowing AOP Annotations
- Step 08 - Exploring Around AOP annotations with a Timer class
- Step 09 - AOP Best Practice - Creating Common Pointcut Definitions
- Step 10 - Creating Track Time Annotation
- Step 11 - Getting Started with Spring AOP - Thank You
### Learning Maven with Spring and Spring Boot
- Step 01 - Introduction to Maven
- Step 02 - Creating a Spring Boot Project with Maven
- Step 03 - Exploring Maven pom.xml for Spring Boot Project
- Step 04 - Exploring Maven Parent Pom for Spring Boot Project
- Step 05 - Exploring Maven Further
- Step 06 - Exploring Maven Build Lifecycle with a Spring Boot Project
- Step 07 - How does Maven Work?
- Step 08 - Playing with Maven Commands
- Step 09 - How are Spring Projects Versioned?
### Learning Gradle with Spring and Spring Boot
- Step 01 - Getting Started with Gradle
- Step 02 - Creating a Spring Boot Project with Gradle
- Step 03 - Exploring Gradle Build and Settings Files
- Step 04 - Exploring Gradle Plugins for Java and Spring Boot
- Step 05 - Maven or Gradle - Which one to use for Spring Boot Projects?
### Learning Docker with Spring and Spring Boot
- Step 01 - Getting Started with Docker
- Step 02 - Understanding Docker Fundamentals
- Step 03 - Understanding How Docker Works
- Step 04 - Understanding Docker Terminology
- Step 05 - Creating Docker Image for a Spring Boot Project - Dockerfile
- Step 06 - Building Spring Boot Docker Image using Multi Stage Dockerfile
- Step 07 - Building Spring Boot Docker Image - Optimizing Dockerfile
- Step 08 - Building Docker Image with Spring Boot Maven Plugin
- Step 09 - Quick Review of Docker with Spring Boot
### Getting Started with Cloud and AWS
- Step 02 - Introduction to Cloud and AWS - Advantages
- Step 03 - Creating Your AWS Account
- Step 04 - Creating Your First IAM User
- Step 05 - Understanding the Need for Regions and Zones
- Step 06 - Exploring Regions and Availability Zones in AWS
### Getting Started with Compute Services in AWS
- Step 01 - Getting Started with EC2 - Virtual Servers in AWS
- Step 02 - Demo - Creating Virtual Machines with Amazon EC2
- Step 02z - Demo - Setting up a Web Server in an Amazon EC2 Instance
- Step 03 - Quick Review of Important EC2 Concepts
- Step 04 - Exploring IaaS vs PaaS - Cloud Computing with AWS
- Step 05 - Getting Started with AWS Elastic Beanstalk
- Step 06 - Demo - Setting up Web Application with AWS Elastic Beanstalk
- Step 07 - Demo - Playing with AWS Elastic Beanstalk
- Step 08 - Understanding the Need for Docker and Containers
- Step 09 - Exploring Container Orchestration in AWS
- Step 10 - Demo - Setting up ECS Cluster with AWS Fargate
- Step 11 - Demo - Playing with Amazon ECS
- Step 12 - Getting Started with Serverless in AWS - AWS Lambda
- Step 13 - Demo - Creating Your First Lambda Function
- Step 14 - Demo - Playing with Lambda Functions
- Step 15 - Cloud Computing in AWS - Quick Review of Compute Services
### Deploying Spring Boot Applications to AWS
- Step 01 - Deploying Hello World Spring Boot App to AWS
- Step 02 - Exploring AWS Elastic Beanstalk - Your First Spring Boot App in AWS
- Step 03 - Running Spring Boot REST API with MySQL Database as Docker Container
- Step 04 - Deploying Spring Boot REST API with MySQL to AWS Elastic Beanstalk and RDS
- Step 05 - Exploring AWS Elastic Beanstalk and Amazon RDS - Spring Boot REST API
- Step 06 - Exploring Spring Boot and React Full Stack App
- Step 07 - Deploying Full Stack Spring Boot REST API to AWS Elastic Beanstalk
- Step 08 - Deploying Full Stack React App to Amazon S3
### Introduction to Functional Programming with Java
- Step 00 - Introduction to Functional Programming - Overview
- Step 01 - Getting Started with Functional Programming with Java
- Step 02 - Writing Your First Java Functional Program
- Step 03 - Improving Java Functional Program with filter
- Step 04 - Using Lambda Expression to enhance your Functional Program
- Step 05 - Do Functional Programming Exercises with Streams, Filters and Lambdas
- Step 06 - Using map in Functional Programs - with Exercises
- Step 07 - Understanding Optional class in Java
- Step 08 - Quick Review of Functional Programming Basics
### Congratulations - Master Spring Framework and Spring Boot
- Congratulations - Master Spring Framework and Spring Boot
| 1 |
code4craft/webmagic | A scalable web crawler framework for Java. | crawler framework java scraping | 
[Readme in Chinese](https://github.com/code4craft/webmagic/tree/master/README-zh.md)
[](https://maven-badges.herokuapp.com/maven-central/us.codecraft/webmagic-parent/)
[](https://www.apache.org/licenses/LICENSE-2.0.html)
[](https://travis-ci.org/code4craft/webmagic)
>A scalable crawler framework. It covers the whole lifecycle of crawler: downloading, url management, content extraction and persistent. It can simplify the development of a specific crawler.
## Features:
* Simple core with high flexibility.
* Simple API for html extracting.
* Annotation with POJO to customize a crawler, no configuration.
* Multi-thread and Distribution support.
* Easy to be integrated.
## Install:
Add dependencies to your pom.xml:
```xml
<dependency>
<groupId>us.codecraft</groupId>
<artifactId>webmagic-core</artifactId>
<version>${webmagic.version}</version>
</dependency>
<dependency>
<groupId>us.codecraft</groupId>
<artifactId>webmagic-extension</artifactId>
<version>${webmagic.version}</version>
</dependency>
```
WebMagic use slf4j with slf4j-log4j12 implementation. If you customized your slf4j implementation, please exclude slf4j-log4j12.
```xml
<exclusions>
<exclusion>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
</exclusion>
</exclusions>
```
## Get Started:
### First crawler:
Write a class implements PageProcessor. For example, I wrote a crawler of github repository infomation.
```java
public class GithubRepoPageProcessor implements PageProcessor {
private Site site = Site.me().setRetryTimes(3).setSleepTime(1000);
@Override
public void process(Page page) {
page.addTargetRequests(page.getHtml().links().regex("(https://github\\.com/\\w+/\\w+)").all());
page.putField("author", page.getUrl().regex("https://github\\.com/(\\w+)/.*").toString());
page.putField("name", page.getHtml().xpath("//h1[@class='public']/strong/a/text()").toString());
if (page.getResultItems().get("name")==null){
//skip this page
page.setSkip(true);
}
page.putField("readme", page.getHtml().xpath("//div[@id='readme']/tidyText()"));
}
@Override
public Site getSite() {
return site;
}
public static void main(String[] args) {
Spider.create(new GithubRepoPageProcessor()).addUrl("https://github.com/code4craft").thread(5).run();
}
}
```
* `page.addTargetRequests(links)`
Add urls for crawling.
You can also use annotation way:
```java
@TargetUrl("https://github.com/\\w+/\\w+")
@HelpUrl("https://github.com/\\w+")
public class GithubRepo {
@ExtractBy(value = "//h1[@class='public']/strong/a/text()", notNull = true)
private String name;
@ExtractByUrl("https://github\\.com/(\\w+)/.*")
private String author;
@ExtractBy("//div[@id='readme']/tidyText()")
private String readme;
public static void main(String[] args) {
OOSpider.create(Site.me().setSleepTime(1000)
, new ConsolePageModelPipeline(), GithubRepo.class)
.addUrl("https://github.com/code4craft").thread(5).run();
}
}
```
### Docs and samples:
Documents: [http://webmagic.io/docs/](http://webmagic.io/docs/)
The architecture of webmagic (refered to [Scrapy](http://scrapy.org/))
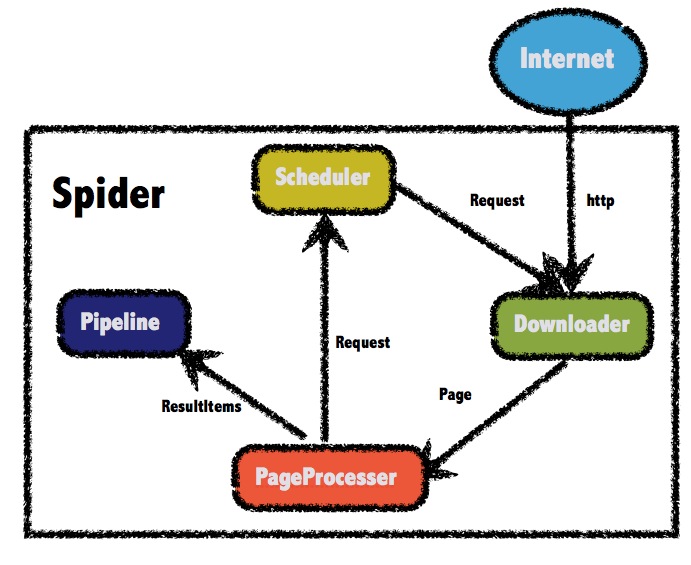
There are more examples in `webmagic-samples` package.
### License:
Licensed under [Apache 2.0 license](http://opensource.org/licenses/Apache-2.0)
### Thanks:
To write webmagic, I refered to the projects below :
* **Scrapy**
A crawler framework in Python.
[http://scrapy.org/](http://scrapy.org/)
* **Spiderman**
Another crawler framework in Java.
[http://git.oschina.net/l-weiwei/spiderman](http://git.oschina.net/l-weiwei/spiderman)
### Mail-list:
[https://groups.google.com/forum/#!forum/webmagic-java](https://groups.google.com/forum/#!forum/webmagic-java)
[http://list.qq.com/cgi-bin/qf_invite?id=023a01f505246785f77c5a5a9aff4e57ab20fcdde871e988](http://list.qq.com/cgi-bin/qf_invite?id=023a01f505246785f77c5a5a9aff4e57ab20fcdde871e988)
QQ Group: 373225642 542327088
### Related Project
* <a href="https://github.com/gsh199449/spider" target="_blank">Gather Platform</a>
A web console based on WebMagic for Spider configuration and management.
| 0 |
hyperledger/besu | An enterprise-grade Java-based, Apache 2.0 licensed Ethereum client https://wiki.hyperledger.org/display/besu | besu blockchain ethereum java p2p | # Besu Ethereum Client
[](https://circleci.com/gh/hyperledger/besu/tree/main)
[](https://besu.hyperledger.org/en/latest/?badge=latest)
[](https://bestpractices.coreinfrastructure.org/projects/3174)
[](https://github.com/hyperledger/besu/blob/main/LICENSE)
[](https://discord.gg/hyperledger)
[](https://twitter.com/HyperledgerBesu)
[Download](https://hyperledger.jfrog.io/artifactory/besu-binaries/besu/)
Besu is an Apache 2.0 licensed, MainNet compatible, Ethereum client written in Java.
## Useful Links
* [Besu User Documentation]
* [Besu Issues]
* [Besu Wiki](https://wiki.hyperledger.org/display/BESU/Hyperledger+Besu)
* [How to Contribute to Besu](https://wiki.hyperledger.org/display/BESU/How+to+Contribute)
* [Besu Roadmap & Planning](https://wiki.hyperledger.org/pages/viewpage.action?pageId=24781786)
## Issues
Besu issues are tracked [in the github issues tab][Besu Issues].
See our [guidelines](https://wiki.hyperledger.org/display/BESU/Issues) for more details on searching and creating issues.
If you have any questions, queries or comments, [Besu channel on Hyperledger Discord] is the place to find us.
## Besu Users
To install the Besu binary, follow [these instructions](https://besu.hyperledger.org/public-networks/get-started/install/binary-distribution).
## Besu Developers
* [Contributing Guidelines]
* [Coding Conventions](https://wiki.hyperledger.org/display/BESU/Coding+Conventions)
* [Command Line Interface (CLI) Style Guide](https://wiki.hyperledger.org/display/BESU/Besu+CLI+Style+Guide)
* [Besu User Documentation] for running and using Besu
### Development
Instructions for how to get started with developing on the Besu codebase. Please also read the
[wiki](https://wiki.hyperledger.org/display/BESU/Pull+Requests) for more details on how to submit a pull request (PR).
* [Checking Out and Building](https://wiki.hyperledger.org/display/BESU/Building+from+source)
* [Running Developer Builds](https://wiki.hyperledger.org/display/BESU/Building+from+source#running-developer-builds)
* [Code Coverage](https://wiki.hyperledger.org/display/BESU/Code+coverage)
* [Logging](https://wiki.hyperledger.org/display/BESU/Logging) or the [Documentation's Logging section](https://besu.hyperledger.org/public-networks/how-to/monitor/logging)
## Release Notes
[Release Notes](CHANGELOG.md)
## Special thanks
YourKit for providing us with a free profiler open source license.
YourKit supports open source projects with innovative and intelligent tools
for monitoring and profiling Java and .NET applications.
YourKit is the creator of <a href="https://www.yourkit.com/java/profiler/">YourKit Java Profiler</a>,
<a href="https://www.yourkit.com/.net/profiler/">YourKit .NET Profiler</a>,
and <a href="https://www.yourkit.com/youmonitor/">YourKit YouMonitor</a>.

[Besu Issues]: https://github.com/hyperledger/besu/issues
[Besu User Documentation]: https://besu.hyperledger.org
[Besu channel on Hyperledger Discord]: https://discord.gg/hyperledger
[Contributing Guidelines]: CONTRIBUTING.md
| 0 |
rapidoid/rapidoid | Rapidoid - Extremely Fast, Simple and Powerful Java Web Framework and HTTP Server! | full-stack high-performance java rapidoid reactive restful web-framework webframework | Rapidoid - Simple. Powerful. Secure. Fast!
========
Rapidoid is an extremely fast HTTP server and modern Java web framework / application container, with a strong focus on high productivity and high performance.
# Documentation, examples, community support
Please visit the official site:
[http://www.rapidoid.org/](http://www.rapidoid.org/)
# Apache License v2
Rapidoid is released under the liberal Apache Public License v2, so it is free to use for both commercial and non-commercial projects.
# Roadmap
* Better documentation (work in progress - as always)
* Swagger / OpenAPI support
# Contributing
1. Fork (and then `git clone https://github.com/<your-username-here>/rapidoid.git`).
2. Make your changes
3. Commit your changes (`git commit -am "Description of contribution"`).
4. Push to GitHub (`git push`).
5. Open a Pull Request.
6. Please sign the CLA.
7. Thank you for your contribution! Wait for a response...
| 0 |
killme2008/aviatorscript | A high performance scripting language hosted on the JVM. | aviator aviatorscript expression-evaluator java jvm-languages scripting-language | # AviatorScript
[](https://travis-ci.org/killme2008/aviatorscript)
[](https://search.maven.org/search?q=g:com.googlecode.aviator%20AND%20aviator)
[📖 English Documentation](README-EN.md) | 📖 中文文档
----------------------------------------
`AviatorScript` 是一门高性能、轻量级寄宿于 JVM (包括 Android 平台)之上的脚本语言。
# 特性介绍
1. 支持数字、字符串、正则表达式、布尔值、正则表达式等[基本类型](https://www.yuque.com/boyan-avfmj/aviatorscript/lvabnw),完整支持所有 Java 运算符及优先级等。
2. [函数](https://www.yuque.com/boyan-avfmj/aviatorscript/gl2p0q)是一等公民,支持[闭包和函数式编程](https://www.yuque.com/boyan-avfmj/aviatorscript/ksghfc)。
3. 内置 [bigint](https://www.yuque.com/boyan-avfmj/aviatorscript/lvabnw#a0Ifn)/[decimal](https://www.yuque.com/boyan-avfmj/aviatorscript/lvabnw#QbV7z) 类型用于大整数和高精度运算,支持[运算符重载](https://www.yuque.com/boyan-avfmj/aviatorscript/ydllav#5hq4k)得以让这些类型使用普通的算术运算符 `+-*/ `参与运算。
4. 完整的脚本语法支持,包括多行数据、条件语句、循环语句、词法作用域和异常处理等。
5. [函数式编程](https://www.yuque.com/boyan-avfmj/aviatorscript/ksghfc)结合 [Sequence 抽象](https://www.yuque.com/boyan-avfmj/aviatorscript/yc4l93),便捷处理任何集合。
6. 轻量化的[模块系统](https://www.yuque.com/boyan-avfmj/aviatorscript/rqra81)。
7. 多种方式,方便地[调用 Java 方法](https://www.yuque.com/boyan-avfmj/aviatorscript/xbdgg2),完整支持 Java [脚本 API](https://www.yuque.com/boyan-avfmj/aviatorscript/bds23b)(方便从 Java 调用脚本)。
8. 丰富的定制选项,可作为安全的语言沙箱和全功能语言使用。
9. 轻量化,高性能,ASM 模式下通过直接将脚本翻译成 JVM 字节码,[解释模式](https://www.yuque.com/boyan-avfmj/aviatorscript/ok8agx)可运行于 Android 等非标 Java 平台。
10. 支持编译后表达式序列化,方便缓存加速等。
使用场景包括:
1. 规则判断及规则引擎
2. 公式计算
3. 动态脚本控制
4. 集合数据 ELT 等
……
**推荐使用版本 5.2.6 及以上**
# News
* [5.4.1](https://github.com/killme2008/aviatorscript/releases/tag/aviator-5.4.1),修复递归函数无法工作的 bug,修复函数无法序列化的 bug 等。
* [5.4.0](https://github.com/killme2008/aviatorscript/releases/tag/aviator-5.4.0),修复 `elsif` 语法解析错误,增加编译表达式序列化支持([例子](https://github.com/killme2008/aviatorscript/blob/master/src/test/java/com/googlecode/aviator/example/SerializeExample.java))等。
* [5.3.3](https://github.com/killme2008/aviatorscript/releases/tag/aviator-5.3.3),修复潜在内存泄露、变量捕获错误等 Bug。
# Dependency
```xml
<dependency>
<groupId>com.googlecode.aviator</groupId>
<artifactId>aviator</artifactId>
<version>{version}</version>
</dependency>
```
可以在 [search.maven.org](https://search.maven.org/search?q=g:com.googlecode.aviator%20AND%20a:aviator&core=gav) 查看可用的版本。
# 快速开始
1. 下载 [aviator](https://raw.githubusercontent.com/killme2008/aviator/master/bin/aviator) shell 到某个目录(最好是在系统的 `PATH` 环境变量内),比如 `~/bin/aviator`:
```sh
$ wget https://raw.githubusercontent.com/killme2008/aviator/master/bin/aviator
$ chmod u+x aviator
```
2. 执行 `aviator` 命令,将自动下载最新文档版本 aviator jar 到 `~/.aviatorscript` 下的安装目录并运行:
```sh
$ aviator
Downloading AviatorScript now...
% Total % Received % Xferd Average Speed Time Time Time Current
Dload Upload Total Spent Left Speed
100 153 100 153 0 0 111 0 0:00:01 0:00:01 --:--:-- 111
100 1373k 100 1373k 0 0 689k 0 0:00:01 0:00:01 --:--:-- 689k
Usage: java com.googlecode.aviator.Main [file] [args]
: java com.googlecode.aviator.Main -e [script]
: java com.googlecode.aviator.Main -v
```
3. 将下面这个脚本保存为文件 `hello.av`:
```js
p("Hello, AviatorScript!");
let a = tuple(1, 2, 3, 4, 5);
p("sum of a is: " + reduce(a, +, 0));
let date = new java.util.Date();
p("The year is: "+ getYear(date));
p("The month is: #{getMonth(date)}");
```
一个更复杂的计算器例子(求值算术表达式字符串)参见[calculator.av](https://github.com/killme2008/aviatorscript/blob/master/examples/calculator.av)。
4. 执行脚本:
```sh
$ aviator hello.av
Hello, AviatorScript!
sum of a is: 15
The year is: 120
The month is: 3
```
更详细的请阅读[用户指南](https://www.yuque.com/boyan-avfmj/aviatorscript/cpow90)。
# Links
* Releases: <https://github.com/killme2008/aviator/releases>
* Documents: <https://www.yuque.com/boyan-avfmj/aviatorscript>
* Changelog: <https://www.yuque.com/boyan-avfmj/aviatorscript/bggwx2>
* Javadoc: <http://fnil.net/aviator/apidocs/>
* Spring boot rule: <https://github.com/mengxiangrui007/spring-boot-rule-jsr94>
* Idea plugin: <https://github.com/yanchangyou/aviatorscript-ideaplugin>
| 0 |
elastic/logstash | Logstash - transport and process your logs, events, or other data | etl-framework java jruby logging real-time-processing streaming | # Logstash
Logstash is part of the [Elastic Stack](https://www.elastic.co/products) along with Beats, Elasticsearch and Kibana. Logstash is a server-side data processing pipeline that ingests data from a multitude of sources simultaneously, transforms it, and then sends it to your favorite "stash." (Ours is Elasticsearch, naturally.). Logstash has over 200 plugins, and you can write your own very easily as well.
For more info, see <https://www.elastic.co/products/logstash>
## Documentation and Getting Started
You can find the documentation and getting started guides for Logstash
on the [elastic.co site](https://www.elastic.co/guide/en/logstash/current/getting-started-with-logstash.html)
For information about building the documentation, see the README in https://github.com/elastic/docs
## Downloads
You can download officially released Logstash binaries, as well as debian/rpm packages for the
supported platforms, from [downloads page](https://www.elastic.co/downloads/logstash).
## Need Help?
- [Logstash Forum](https://discuss.elastic.co/c/logstash)
- [Logstash Documentation](https://www.elastic.co/guide/en/logstash/current/index.html)
- [#logstash on freenode IRC](https://webchat.freenode.net/?channels=logstash)
- [Logstash Product Information](https://www.elastic.co/products/logstash)
- [Elastic Support](https://www.elastic.co/subscriptions)
## Logstash Plugins
Logstash plugins are hosted in separate repositories under the [logstash-plugins](https://github.com/logstash-plugins) github organization. Each plugin is a self-contained Ruby gem which gets published to RubyGems.org.
### Writing your own Plugin
Logstash is known for its extensibility. There are hundreds of plugins for Logstash and you can write your own very easily! For more info on developing and testing these plugins, please see the [working with plugins section](https://www.elastic.co/guide/en/logstash/current/contributing-to-logstash.html)
### Plugin Issues and Pull Requests
**Please open new issues and pull requests for plugins under its own repository**
For example, if you have to report an issue/enhancement for the Elasticsearch output, please do so [here](https://github.com/logstash-plugins/logstash-output-elasticsearch/issues).
Logstash core will continue to exist under this repository and all related issues and pull requests can be submitted here.
## Developing Logstash Core
### Prerequisites
* Install JDK version 11 or 17. Make sure to set the `JAVA_HOME` environment variable to the path to your JDK installation directory. For example `set JAVA_HOME=<JDK_PATH>`
* Install JRuby 9.2.x It is recommended to use a Ruby version manager such as [RVM](https://rvm.io/) or [rbenv](https://github.com/sstephenson/rbenv).
* Install `rake` and `bundler` tool using `gem install rake` and `gem install bundler` respectively.
### RVM install (optional)
If you prefer to use rvm (ruby version manager) to manage Ruby versions on your machine, follow these directions. In the Logstash folder:
```sh
gpg --keyserver hkp://keys.gnupg.net --recv-keys 409B6B1796C275462A1703113804BB82D39DC0E3
\curl -sSL https://get.rvm.io | bash -s stable --ruby=$(cat .ruby-version)
```
### Check Ruby version
Before you proceed, please check your ruby version by:
```sh
$ ruby -v
```
The printed version should be the same as in the `.ruby-version` file.
### Building Logstash
The Logstash project includes the source code for all of Logstash, including the Elastic-Licensed X-Pack features and functions; to run Logstash from source using only the OSS-licensed code, export the `OSS` environment variable with a value of `true`:
``` sh
export OSS=true
```
* Set up the location of the source code to build
``` sh
export LOGSTASH_SOURCE=1
export LOGSTASH_PATH=/YOUR/LOGSTASH/DIRECTORY
```
#### Install dependencies with `gradle` **(recommended)**[^1]
* Install development dependencies
```sh
./gradlew installDevelopmentGems
```
* Install default plugins and other dependencies
```sh
./gradlew installDefaultGems
```
### Verify the installation
To verify your environment, run the following to start Logstash and send your first event:
```sh
bin/logstash -e 'input { stdin { } } output { stdout {} }'
```
This should start Logstash with stdin input waiting for you to enter an event
```sh
hello world
2016-11-11T01:22:14.405+0000 0.0.0.0 hello world
```
**Advanced: Drip Launcher**
[Drip](https://github.com/ninjudd/drip) is a tool that solves the slow JVM startup problem while developing Logstash. The drip script is intended to be a drop-in replacement for the java command. We recommend using drip during development, in particular for running tests. Using drip, the first invocation of a command will not be faster but the subsequent commands will be swift.
To tell logstash to use drip, set the environment variable `` JAVACMD=`which drip` ``.
Example (but see the *Testing* section below before running rspec for the first time):
JAVACMD=`which drip` bin/rspec
**Caveats**
Drip does not work with STDIN. You cannot use drip for running configs which use the stdin plugin.
## Building Logstash Documentation
To build the Logstash Reference (open source content only) on your local
machine, clone the following repos:
[logstash](https://github.com/elastic/logstash) - contains main docs about core features
[logstash-docs](https://github.com/elastic/logstash-docs) - contains generated plugin docs
[docs](https://github.com/elastic/docs) - contains doc build files
Make sure you have the same branch checked out in `logstash` and `logstash-docs`.
Check out `master` in the `docs` repo.
Run the doc build script from within the `docs` repo. For example:
```
./build_docs.pl --doc ../logstash/docs/index.asciidoc --chunk=1 -open
```
## Testing
Most of the unit tests in Logstash are written using [rspec](http://rspec.info/) for the Ruby parts. For the Java parts, we use [junit](https://junit.org). For testing you can use the *test* `rake` tasks and the `bin/rspec` command, see instructions below:
### Core tests
1- To run the core tests you can use the Gradle task:
./gradlew test
or use the `rspec` tool to run all tests or run a specific test:
bin/rspec
bin/rspec spec/foo/bar_spec.rb
Note that before running the `rspec` command for the first time you need to set up the RSpec test dependencies by running:
./gradlew bootstrap
2- To run the subset of tests covering the Java codebase only run:
./gradlew javaTests
3- To execute the complete test-suite including the integration tests run:
./gradlew check
4- To execute a single Ruby test run:
SPEC_OPTS="-fd -P logstash-core/spec/logstash/api/commands/default_metadata_spec.rb" ./gradlew :logstash-core:rubyTests --tests org.logstash.RSpecTests
5- To execute single spec for integration test, run:
./gradlew integrationTests -PrubyIntegrationSpecs=specs/slowlog_spec.rb
Sometimes you might find a change to a piece of Logstash code causes a test to hang. These can be hard to debug.
If you set `LS_JAVA_OPTS="-agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=5005"` you can connect to a running Logstash with your IDEs debugger which can be a great way of finding the issue.
### Plugins tests
To run the tests of all currently installed plugins:
rake test:plugins
You can install the default set of plugins included in the logstash package:
rake test:install-default
---
Note that if a plugin is installed using the plugin manager `bin/logstash-plugin install ...` do not forget to also install the plugins development dependencies using the following command after the plugin installation:
bin/logstash-plugin install --development
## Building Artifacts
Built artifacts will be placed in the `LS_HOME/build` directory, and will create the directory if it is not already present.
You can build a Logstash snapshot package as tarball or zip file
```sh
./gradlew assembleTarDistribution
./gradlew assembleZipDistribution
```
OSS-only artifacts can similarly be built with their own gradle tasks:
```sh
./gradlew assembleOssTarDistribution
./gradlew assembleOssZipDistribution
```
You can also build .rpm and .deb, but the [fpm](https://github.com/jordansissel/fpm) tool is required.
```sh
rake artifact:rpm
rake artifact:deb
```
and:
```sh
rake artifact:rpm_oss
rake artifact:deb_oss
```
## Using a Custom JRuby Distribution
If you want the build to use a custom JRuby you can do so by setting a path to a custom
JRuby distribution's source root via the `custom.jruby.path` Gradle property.
E.g.
```sh
./gradlew clean test -Pcustom.jruby.path="/path/to/jruby"
```
## Project Principles
* Community: If a newbie has a bad time, it's a bug.
* Software: Make it work, then make it right, then make it fast.
* Technology: If it doesn't do a thing today, we can make it do it tomorrow.
## Contributing
All contributions are welcome: ideas, patches, documentation, bug reports,
complaints, and even something you drew up on a napkin.
Programming is not a required skill. Whatever you've seen about open source and
maintainers or community members saying "send patches or die" - you will not
see that here.
It is more important that you are able to contribute.
For more information about contributing, see the
[CONTRIBUTING](./CONTRIBUTING.md) file.
## Footnotes
[^1]: <details><summary>Use bundle instead of gradle to install dependencies</summary>
#### Alternatively, instead of using `gradle` you can also use `bundle`:
* Install development dependencies
```sh
bundle config set --local path vendor/bundle
bundle install
```
* Bootstrap the environment:
```sh
rake bootstrap
```
* You can then use `bin/logstash` to start Logstash, but there are no plugins installed. To install default plugins, you can run:
```sh
rake plugin:install-default
```
This will install the 80+ default plugins which makes Logstash ready to connect to multiple data sources, perform transformations and send the results to Elasticsearch and other destinations.
</details>
| 0 |
yacy/yacy_search_server | Distributed Peer-to-Peer Web Search Engine and Intranet Search Appliance | null | <div align="center">
<h1 align="center">YaCy</h1>
Search Engine Software
[](https://yacy.net)
[](https://community.searchlab.eu/)
[](https://github.com/sponsors/Orbiter)
[](https://www.patreon.com/bePatron?u=185903)
[](https://github.com/yacy/yacy_search_server/actions/workflows/ant-build-selfhosted.yaml)
[](https://yacy.net/download_installation/)



</div>
## What is YaCy?
YaCy is a full search engine application containing a server hosting a search index,
a web application to provide a nice user front-end for searches and index creation
and a production-ready web crawler with a scheduler to keep a search index fresh.
YaCy search portals can also be placed in an intranet environment, making
it a replacement for commercial enterprise search solutions. A network
scanner makes it easy to discover all available HTTP, FTP and SMB servers.
Running a personal Search Engine is a great tool for privacy; indeed YaCy
was created with the privacy aspect as priority motivation for the project.
You can also use YaCy with a customized search page in your own web applications.
## Large-Scale Web Search with a Peer-to-Peer Network
Each YaCy peer can be part of a large search network where search indexes can be
exchanged with other YaCy installation over a built-in peer-to-peer network protocol.
This is the default operation that enables new users to instantly access
a large-scale search cluster, operated only by YaCy users.
You can opt-out from the YaCy cluster operation by choosing a different operation
mode in the web interface. You can also opt-out from the network in individual searches,
turning the use of YaCy a completely privacy-aware tool - in this operation mode search
results are computed from the local index only.
## Installation
We recommend to compile YaCy yourself and install it from the git sources.
Pre-compiled YaCy packages exist but are not generated on a regular basis.
Automaticaly built latest developer release is available at
[release.yacy.net](https://release.yacy.net/).
To get a ready-to-run production package, run YaCy from Docker.
### Compile and run YaCy from git sources
You need Java 11 or later to run YaCy and ant to build YaCy.
This would install the requirements on debian:
```
sudo apt-get install openjdk-11-jdk-headless ant
```
Then clone the repository and build the application:
```
git clone --depth 1 https://github.com/yacy/yacy_search_server.git
cd yacy_search_server
ant clean all
```
To start YaCy, run
```
./startYACY.sh
```
The administration interface is then available in your web browser at `http://localhost:8090`.
Some of the web pages are protected and need an administration account; these pages are usually
also available without a password from the localhost, but remote access needs a log-in.
The default admin account name is `admin` and the default password is `yacy`.
Please change it after installation using the ``http://<server-address>:8090/ConfigAccounts_p.html`` service.
Stop YaCy on the console with
```
./stopYACY.sh
```
### Run YaCy using Docker
The Official YaCy Image is `yacy/yacy_search_server:latest`. It is hosted on Dockerhub at [https://hub.docker.com/r/yacy/yacy_search_server](https://hub.docker.com/r/yacy/yacy_search_server)
To install YaCy in intel-based environments, run:
```
docker run -d --name yacy_search_server -p 8090:8090 -p 8443:8443 -v yacy_search_server_data:/opt/yacy_search_server/DATA --restart unless-stopped --log-opt max-size=200m --log-opt max-file=2 yacy/yacy_search_server:latest
```
then open http://localhost:8090 in your web-browser.
For building Docker image from latest sources, see [docker/Readme.md](docker/Readme.md).
## Help develop YaCy
- clone https://github.com/yacy/yacy_search_server.git using build-in Eclipse features (File -> Import -> Git)
- or download source from this site (download button "Code" -> download as Zip -> and unpack)
- Open Help -> Eclipse Marketplace -> Search for "ivy" -> Install "Apache IvyDE"
- right-click on the YaCy project in the package explorer -> Ivy -> resolve
This will build YaCy in Eclipse. To run YaCy:
- Package Explorer -> YaCy: navigate to source -> net.yacy
- right-click on yacy.java -> Run as -> Java Application
Join our development community, got to https://community.searchlab.eu
Send pull requests to https://github.com/yacy/yacy_search_server
## APIs and attaching software
YaCy has many built-in interfaces, and they are all based on HTTP/XML and
HTTP/JSON. You can discover these interfaces if you notice the orange "API" icon in
the upper right corner of some web pages in the YaCy web interface. Click it, and
you will see the XML/JSON version of the respective webpage.
You can also use the shell script provided in the /bin subdirectory.
The shell scripts also call the YaCy web interface. By cloning some of those
scripts you can easily create more shell API access methods.
## License
This project is available as open source under the terms of the GPL 2.0 or later. However, some elements are being licensed under GNU Lesser General Public License. For accurate information, please check individual files. As well as for accurate information regarding copyrights.
The (GPLv2+) source code used to build YaCy is distributed with the package (in /source and /htroot).
## Contact
[Visit the international YaCy forum](https://community.searchlab.eu)
where you can start a discussion there in your own language.
Questions and requests for paid customization and integration into enterprise solutions.
can be sent to the maintainer, Michael Christen per e-mail (at mc@yacy.net)
with a meaningful subject including the word 'YaCy' to prevent it getting stuck in the spam filter.
- Michael Peter Christen
| 0 |
sagframe/sagacity-sqltoy | Java真正智慧的ORM框架,融合JPA功能和最佳的sql编写及查询模式、独创的缓存翻译、最优化的分页、并提供无限层级分组汇总、同比环比、行列转换、树形排序汇总、sql自适配不同数据库、分库分表、多租户、数据加解密、脱敏以及面向复杂业务和大规模数据分析等痛点、难点问题项目实践经验分享的一站式解决方案! | orm sagacity sagacity-orm sagacity-sqltoy sql sql-orm sqltoy sqltoy-orm sqltoy-sql | <p align="center">
<a target="_blank" href="LICENSE"><img src="https://img.shields.io/:license-Apache%202.0-blue.svg"></a>
<a target="_blank" href="https://github.com/sagframe/sagacity-sqltoy"><img src="https://img.shields.io/github/stars/sagframe/sagacity-sqltoy.svg?style=social"/></a>
<a target="_blank" href="https://gitee.com/sagacity/sagacity-sqltoy"><img src="https://gitee.com/sagacity/sagacity-sqltoy/badge/star.svg?theme=white" /></a>
<a target="_blank" href="https://github.com/sagframe/sagacity-sqltoy/releases"><img src="https://img.shields.io/github/v/release/sagframe/sagacity-sqltoy?logo=github"></a>
<a href="https://mvnrepository.com/artifact/com.sagframe/sagacity-sqltoy">
<img alt="maven" src="https://img.shields.io/maven-central/v/com.sagframe/sagacity-sqltoy?style=flat-square">
</a>
</p>
# WORD版详细文档(完整)
## 请见:docs/睿智平台SqlToy5.6 使用手册.doc
## xml中sql查询完整配置
https://github.com/sagframe/sqltoy-online-doc/blob/master/docs/sqltoy/search.md
# [gitee地址](https://gitee.com/sagacity/sagacity-sqltoy)
# [sqltoy Lambda](https://gitee.com/gzghde/sqltoy-plus)
# [sqltoy管理系统脚手架](https://gitee.com/momoljw/sss-rbac-admin)
# 范例演示项目
## 快速集成演示项目
* https://gitee.com/sagacity/sqltoy-helloworld
* 阅读其readme.md学习
## 快速上手功能演示项目
* https://github.com/sagframe/sqltoy-quickstart
* 阅读其readme.md学习
## POJO和DTO 严格分层演示项目
* https://github.com/sagframe/sqltoy-strict
## sharding分库分表演示
* https://github.com/sagframe/sqltoy-showcase/tree/master/trunk/sqltoy-sharding
## dynamic-datasource多数据源范例
* https://gitee.com/sagacity/sqltoy-showcase/tree/master/trunk/sqltoy-dynamic-datasource
## nosql演示(mongo和elasticsearch)
* https://github.com/sagframe/sqltoy-showcase/tree/master/trunk/sqltoy-nosql
## sqltoy基于xml配置演示
* https://github.com/sagframe/sqltoy-showcase/tree/master/trunk/sqltoy-showcase
# QQ 交流群:531812227
# 码云地址: https://gitee.com/sagacity/sagacity-sqltoy
# 最新版本
* 5.6.4 LTS (jdk17+/springboot3.x)/5.6.4.jre8 (解耦跟spring的强绑定关系,兼容5.2.x/5.3.x版本) 发版日期: 2024-4-3
```xml
<dependency>
<groupId>com.sagframe</groupId>
<artifactId>sagacity-sqltoy-spring-starter</artifactId>
<!-- solon 适配版本 <artifactId>sagacity-sqltoy-solon-plugin</artifactId> -->
<!-- jdk8 对应的版本号为:5.6.4.jre8 -->
<version>5.6.4</version>
</dependency>
```
* 5.2.103 LTS (jdk1.8+) 发版日期: 2024-4-3
# 历史版本(EOL 不再维护) 建议升级到5.6.x版本
* 5.1.81 发版日期: 2023-12-06
* 4.20.87(兼容所有之前版本) 发版日期: 2023-12-05
# 1. 前言
## 1.1 sqltoy-orm是什么
sqltoy-orm是JPA和超强查询的融合体,是简单业务、大型SaaS化多租户ERP、大数据分析多种类型项目实践过程的总结和分享。
### JPA部分
* 类似JPA的对象化CRUD、对象级联加载和新增、更新
* 支持通过POJO生成DDL以及直接向数据库创建表
* 强化update操作,提供弹性字段修改能力,不同于hibernate先load后修改,而是一次数据库交互完成修改,确保了高并发场景下数据的准确性
* 改进了级联修改,提供了先删除或者先置无效,再覆盖的操作选项
* 增加了updateFetch、updateSaveFetch功能,强化针对强事务高并发场景的处理,类似库存台账、资金台账,实现一次数据库交互,完成锁查询、不存在则插入、存在则修改,并返回修改后的结果
* 增加了树结构封装,便于统一不同数据库树型结构数据的递归查询
* 支持分库分表、支持多种主键策略(额外支持基于redis的产生特定规则的业务主键)、加密存储、数据版本校验
* 提供了公共属性赋值(创建人、修改人、创建时间、修改时间、租户)、扩展类型处理等
* 提供了多租户统一过滤和赋值、提供了数据权限参数带入和越权校验
### 查询部分
* 极为直观的sql编写方式,便于从客户端<-->代码 双向快速迁移,便于后期变更维护
* 支持缓存翻译、反向缓存匹配key代替like模糊查询
* 提供了跨数据库支持能力:不同数据库的函数自动转换适配,多方言sql根据实际环境自动匹配、多数据库同步测试,大幅提升了产品化能力
* 提供了取top记录、随机记录等特殊场景的查询功能
* 提供了最强大的分页查询机制:1)自动优化count语句;2)提供基于缓存的分页优化,避免每次都执行count查询;3)提供了独具特色的快速分页;4)提供了并行分页
* 提供了分库分表能力
* 提供了在管理类项目中极为价值的:分组汇总计算、行列转换(行转列、列转行)、同比环比、树形排序、树形汇总 相关算法自然集成
* 提供了基于查询的层次化数据结构封装
* 提供了大量辅助功能:数据脱敏、格式化、条件参数预处理等
### 支持多种数据库
* 常规的mysql、oracle、db2、postgresql、 sqlserver、dm、kingbase、sqlite、h2、 oceanBase、polardb、gaussdb、tidb、oscar(神通)、瀚高
* 支持分布式olap数据库: clickhouse、StarRocks、greenplum、impala(kudu)
* 支持elasticsearch、mongodb
* 所有基于sql和jdbc 各类数据库查询
## 1.2 sqltoy-orm 发展轨迹
* 2007~2008年,做农行的一个管理类项目,因查询统计较多,且查询条件多而且经常要增加条件,就不停想如何快速适应这种变化,一个比较偶然的灵感发现了比mybatis强无数倍的动态sql写法,并作为hibernate jpa 查询方面的补充,收到了极为震撼的开发体验。可以看写于2009年的一篇博文: https://blog.csdn.net/iteye_2252/article/details/81683940
* 2008~2012年,因一直做金融类企业项目,所面对的数据规模基本上是千万级别的,因此sqltoy一直围绕jpa进行sql查询增强,期间已经集成了缓存翻译、快速分页、行列旋转等比其他框架更具特色的查询特性。
* 2013~2014年,因为了避免让开发者在项目中同时使用两种技术,因此在sqltoy中实现了基于对象的crud功能,形成了完整的sqltoy-orm框架。
* 2014~2017年, 在负责拉卡拉大数据平台过程中,对sqltoy进行了大幅重构,实现了底层结构的合理化,在拉卡拉CRM和日均千万累计达百亿级别的大数据平台上得到了强化和检验。
* 2018~至今, 在建设SaaS化多租户ERP复杂场景下和flink-cdc结合MPP数据库的实时数仓过程中得到了充分锤炼,sqltoy已经非常完善可靠,开始开源跟大家一起分享和共建!
# 2. 快速特点说明
## 2.1 对象操作跟jpa类似并有针对性加强(包括级联)
* 通过quickvo工具从数据库生成对应的POJO,注入sqltoy自带的LightDao完成全部操作
```java
//三步曲:1、quickvo生成pojo,2、完成yml配置;3、service中注入dao(无需自定义各种dao)
@Autowired
LightDao lightDao;
StaffInfoVO staffInfo = new StaffInfoVO();
//保存
lightDao.save(staffInfo);
//删除
lightDao.delete(new StaffInfoVO("S2007"));
//public Long update(Serializable entity, String... forceUpdateProps);
// 这里对photo 属性进行强制修改,其他为null自动会跳过
lightDao.update(staffInfo, "photo");
//深度修改,不管是否null全部字段修改
lightDao.updateDeeply(staffInfo);
List<StaffInfoVO> staffList = new ArrayList<StaffInfoVO>();
StaffInfoVO staffInfo = new StaffInfoVO();
StaffInfoVO staffInfo1 = new StaffInfoVO();
staffList.add(staffInfo);
staffList.add(staffInfo1);
//批量保存或修改
lightDao.saveOrUpdateAll(staffList);
//批量保存
lightDao.saveAll(staffList);
...............
lightDao.loadByIds(StaffInfoVO.class,"S2007")
//唯一性验证
lightDao.isUnique(staffInfo, "staffCode");
```
## 2.2 支持代码中对象查询
* sqltoy 中统一的规则是代码中可以直接传sql也可以是对应xml文件中的sqlId
```java
/**
* @todo 通过对象传参数,简化paramName[],paramValue[] 模式传参
* @param <T>
* @param sqlOrNamedSql 可以是具体sql也可以是对应xml中的sqlId
* @param entity 通过对象传参数,并按对象类型返回结果
*/
public <T extends Serializable> List<T> findBySql(final String sqlOrNamedSql, final T entity);
```
* 基于对象单表查询,并带缓存翻译
```java
public Page<StaffInfoVO> findStaff(Page<StaffInfoVO> pageModel, StaffInfoVO staffInfoVO) {
// sql可以直接在代码中编写,复杂sql建议在xml中定义
// 单表entity查询场景下sql字段可以写成java类的属性名称
return findPageEntity(pageModel,StaffInfoVO.class, EntityQuery.create()
.where("#[staffName like :staffName]#[and createTime>=:beginDate]#[and createTime<=:endDate]")
.values(staffInfoVO)
// 字典缓存必须要设置cacheType
// 单表对象查询需设置keyColumn构成select keyColumn as column模式
.translates(new Translate("dictKeyName").setColumn("sexTypeName").setCacheType("SEX_TYPE")
.setKeyColumn("sexType"))
.translates(new Translate("organIdName").setColumn("organName").setKeyColumn("organId")));
}
```
* 对象式查询后修改或删除
```java
//演示代码中非直接sql模式设置条件模式进行记录修改
public Long updateByQuery() {
return lightDao.updateByQuery(StaffInfoVO.class,
EntityUpdate.create().set("createBy", "S0001")
.where("staffName like ?").values("张"));
}
//代码中非直接sql模式设置条件模式进行记录删除
lightDao.deleteByQuery(StaffInfoVO.class, EntityQuery.create().where("status=?").values(0));
```
## 2.2 极致朴素的sql编写方式(本质规律的发现和抽象)
* sqltoy 的写法(一眼就看明白sql的本意,后面变更调整也非常便捷,copy到数据库客户端里稍作调整即可执行)
* sqltoy条件组织原理很简单: 如 #[order_id=:orderId] 等于if(:orderId<>null) sql.append(order_id=:orderId);#[]内只要有一个参数为null即剔除
* 支持多层嵌套:如 #[and t.order_id=:orderId #[and t.order_type=:orderType]]
* 条件判断保留#[@if(:param>=xx ||:param<=xx1) sql语句] 这种@if()高度灵活模式,为特殊复杂场景下提供万能钥匙
```xml
//1、 条件值处理跟具体sql分离
//2、 将条件值前置通过filters 定义的通用方法加工规整(大多数是不需要额外处理的)
<sql id="show_case">
<filters>
<!-- 参数statusAry只要包含-1(代表全部)则将statusAry设置为null不参与条件检索 -->
<eq params="statusAry" value="-1" />
</filters>
<value><![CDATA[
select *
from sqltoy_device_order_info t
where #[t.status in (:statusAry)]
#[and t.ORDER_ID=:orderId]
#[and t.ORGAN_ID in (:authedOrganIds)]
#[and t.STAFF_ID in (:staffIds)]
#[and t.TRANS_DATE>=:beginAndEndDate[0]]
#[and t.TRANS_DATE<:beginAndEndDate[1]]
]]>
</value>
</sql>
```
* mybatis同样的功能的写法
```xml
<select id="show_case" resultMap="BaseResultMap">
select *
from sqltoy_device_order_info t
<where>
<if test="statusAry!=null">
and t.status in
<foreach collection="status" item="statusAry" separator="," open="(" close=")">
#{status}
</foreach>
</if>
<if test="orderId!=null">
and t.ORDER_ID=#{orderId}
</if>
<if test="authedOrganIds!=null">
and t.ORGAN_ID in
<foreach collection="authedOrganIds" item="order_id" separator="," open="(" close=")">
#{order_id}
</foreach>
</if>
<if test="staffIds!=null">
and t.STAFF_ID in
<foreach collection="staffIds" item="staff_id" separator="," open="(" close=")">
#{staff_id}
</foreach>
</if>
<if test="beginDate!=null">
and t.TRANS_DATE>=#{beginDate}
</if>
<if test="endDate!=null">
and t.TRANS_DATE<#{endDate}
</if>
</where>
</select>
```
## 2.3 天然防止sql注入,执行过程:
```xml
假设sql语句如下
select *
from sqltoy_device_order_info t
where #[t.ORGAN_ID in (:authedOrganIds)]
#[and t.TRANS_DATE>=:beginDate]
#[and t.TRANS_DATE<:endDate]
java调用过程:
lightDao.find(sql, MapKit.keys("authedOrganIds","beginDate", "endDate").values(authedOrganIdAry,beginDate,null), DeviceOrderInfoVO.class);
最终执行的sql是这样的:
select *
from sqltoy_device_order_info t
where t.ORDER_ID=?
and t.ORGAN_ID in (?,?,?)
and t.TRANS_DATE>=?
然后通过: pst.set(index,value) 设置条件值,不存在将条件直接作为字符串拼接为sql的一部分
```
## 2.4 最强大的分页查询
### 2.4.1 分页特点说明
* 1、快速分页:@fast() 实现先取单页数据然后再关联查询,极大提升速度。
* 2、分页优化器:page-optimize 让分页查询由两次变成1.3~1.5次(用缓存实现相同查询条件的总记录数量在一定周期内无需重复查询)
* 3、sqltoy的分页取总记录的过程不是简单的select count(1) from (原始sql);而是智能判断是否变成:select count(1) from 'from后语句',
并自动剔除最外层的order by
* 4、sqltoy支持并行查询:parallel="true",同时查询总记录数和单页数据,大幅提升性能
* 5、在极特殊情况下sqltoy分页考虑是最优化的,如:with t1 as (),t2 as @fast(select * from table1) select * from xxx
这种复杂查询的分页的处理,sqltoy的count查询会是:with t1 as () select count(1) from table1,
如果是:with t1 as @fast(select * from table1) select * from t1 ,count sql 就是:select count(1) from table1
### 2.4.2 分页sql示例
```xml
<!-- 快速分页和分页优化演示 -->
<sql id="sqltoy_fastPage">
<!-- 分页优化器,通过缓存实现查询条件一致的情况下在一定时间周期内缓存总记录数量,从而无需每次查询总记录数量 -->
<!-- parallel:是否并行查询总记录数和单页数据,当alive-max=1 时关闭缓存优化 -->
<!-- alive-max:最大存放多少个不同查询条件的总记录量; alive-seconds:查询条件记录量存活时长(比如120秒,超过阀值则重新查询) -->
<page-optimize parallel="true" alive-max="100" alive-seconds="120" />
<value><![CDATA[
select t1.*,t2.ORGAN_NAME
-- @fast() 实现先分页取10条(具体数量由pageSize确定),然后再关联
from @fast(select t.*
from sqltoy_staff_info t
where t.STATUS=1
#[and t.STAFF_NAME like :staffName]
order by t.ENTRY_DATE desc
) t1
left join sqltoy_organ_info t2 on t1.organ_id=t2.ORGAN_ID
]]>
</value>
<!-- 这里为极特殊情况下提供了自定义count-sql来实现极致性能优化 -->
<!-- <count-sql></count-sql> -->
</sql>
```
### 2.4.3 分页java代码调用
```java
/**
* 基于对象传参数模式
*/
public void findPageByEntity() {
Page pageModel = new Page();
StaffInfoVO staffVO = new StaffInfoVO();
// 作为查询条件传参数
staffVO.setStaffName("陈");
// 使用了分页优化器
// 第一次调用:执行count 和 取记录两次查询
Page result = lightDao.findPage(pageModel, "sqltoy_fastPage", staffVO);
System.err.println(JSON.toJSONString(result));
// 第二次调用:过滤条件一致,则不会再次执行count查询
//设置为第二页
pageModel.setPageNo(2);
result = lightDao.findPage(pageModel, "sqltoy_fastPage", staffVO);
System.err.println(JSON.toJSONString(result));
}
```
## 2.5 最巧妙的缓存应用,减少表关联查询
* 1、 通过缓存翻译:<translate> 将代码转化为名称,避免关联查询,极大简化sql并提升查询效率
* 2、 通过缓存名称模糊匹配:<cache-arg> 获取精准的编码作为条件,避免关联like 模糊查询
```java
//支持对象属性注解模式进行缓存翻译
@Translate(cacheName = "dictKeyName", cacheType = "DEVICE_TYPE", keyField = "deviceType")
private String deviceTypeName;
@Translate(cacheName = "staffIdName", keyField = "staffId")
private String staffName;
```
```xml
<sql id="sqltoy_order_search">
<!-- 缓存翻译设备类型
cache:具体的缓存定义的名称,
cache-type:一般针对数据字典,提供一个分类条件过滤
columns:sql中的查询字段名称,可以逗号分隔对多个字段进行翻译
cache-indexs:缓存数据名称对应的列,不填则默认为第二列(从0开始,1则表示第二列),
例如缓存的数据结构是:key、name、fullName,则第三列表示全称
-->
<translate cache="dictKeyName" cache-type="DEVICE_TYPE" columns="deviceTypeName" cache-indexs="1"/>
<!-- 员工名称翻译,如果同一个缓存则可以同时对几个字段进行翻译 -->
<translate cache="staffIdName" columns="staffName,createName" />
<filters>
<!-- 反向利用缓存通过名称匹配出id用于精确查询 -->
<cache-arg cache-name="staffIdNameCache" param="staffName" alias-name="staffIds"/>
</filters>
<value>
<![CDATA[
select ORDER_ID,
DEVICE_TYPE,
DEVICE_TYPE deviceTypeName,-- 设备分类名称
STAFF_ID,
STAFF_ID staffName, -- 员工姓名
ORGAN_ID,
CREATE_BY,
CREATE_BY createName -- 创建人名称
from sqltoy_device_order_info t
where #[t.ORDER_ID=:orderId]
#[and t.STAFF_ID in (:staffIds)]
]]>
</value>
</sql>
```
## 2.6 并行查询
* 接口规范
```java
// parallQuery 面向查询(不要用于事务操作过程中),sqltoy提供强大的方法,但是否恰当使用需要使用者做合理的判断
/**
* @TODO 并行查询并返回一维List,有几个查询List中就包含几个结果对象,paramNames和paramValues是全部sql的条件参数的合集
* @param parallQueryList
* @param paramNames
* @param paramValues
*/
public <T> List<QueryResult<T>> parallQuery(List<ParallQuery> parallQueryList, String[] paramNames,
Object[] paramValues);
```
* 使用范例
```java
//定义参数
String[] paramNames = new String[] { "userId", "defaultRoles", "deployId", "authObjType" };
Object[] paramValues = new Object[] { userId, defaultRoles, GlobalConstants.DEPLOY_ID,
SagacityConstants.TempAuthObjType.GROUP };
// 使用并行查询同时执行2个sql,条件参数是2个查询的合集
List<QueryResult<TreeModel>> list = super.parallQuery(
Arrays.asList(
ParallQuery.create().sql("webframe_searchAllModuleMenus").resultType(TreeModel.class),
ParallQuery.create().sql("webframe_searchAllUserReports").resultType(TreeModel.class)),
paramNames, paramValues);
```
## 2.7 最跨数据库
* 1、提供类似hibernate性质的对象操作,自动生成相应数据库的方言。
* 2、提供了最常用的:分页、取top、取随机记录等查询,避免了各自不同数据库不同的写法。
* 3、提供了树形结构表的标准钻取查询方式,代替以往的递归查询,一种方式适配所有数据库。
* 4、sqltoy提供了大量基于算法的辅助实现,最大程度上用算法代替了以往的sql,实现了跨数据库
* 5、sqltoy提供了函数替换功能,比如可以让oracle的语句在mysql或sqlserver上执行(sql加载时将函数替换成了mysql的函数),最大程度上实现了代码的产品化。
<property name="functionConverts" value="default" />
default:SubStr\Trim\Instr\Concat\Nvl 函数;可以参见org.sagacity.sqltoy.plugins.function.Nvl 代码实现
```properties
# 开启sqltoy默认的函数自适配转换函数
spring.sqltoy.functionConverts=default
# 如在mysql场景下同时测试其他类型数据库,验证sql适配不同数据库,主要用于产品化软件
spring.sqltoy.redoDataSources[0]=pgdb
# 也可以自定义函数来实现替换Nvl
# spring.sqltoy.functionConverts=default,com.yourpackage.Nvl
# 启用框架自带Nvl、Instr
# spring.sqltoy.functionConverts=Nvl,Instr
# 启用自定义Nvl、Instr
# spring.sqltoy.functionConverts=com.yourpackage.Nvl,com.yourpackage.Instr
```
* 6、通过sqlId+dialect模式,可针对特定数据库写sql,sqltoy根据数据库类型获取实际执行sql,顺序为:
dialect_sqlId->sqlId_dialect->sqlId,
如数据库为mysql,调用sqlId:sqltoy_showcase,则实际执行:sqltoy_showcase_mysql
```xml
<sql id="sqltoy_showcase">
<value>
<![CDATA[
select * from sqltoy_user_log t
where t.user_id=:userId
]]>
</value>
</sql>
<!-- sqlId_数据库方言(小写) -->
<sql id="sqltoy_showcase_mysql">
<value>
<![CDATA[
select * from sqltoy_user_log t
where t.user_id=:userId
]]>
</value>
</sql>
```
## 2.8 提供行列转换、分组汇总、同比环比、树排序汇总等
* 水果销售记录表
品类|销售月份|销售笔数|销售数量(吨)|销售金额(万元)
----|-------|-------|----------|------------
苹果|2019年5月|12 | 2000|2400
苹果|2019年4月|11 | 1900|2600
苹果|2019年3月|13 | 2000|2500
香蕉|2019年5月|10 | 2000|2000
香蕉|2019年4月|12 | 2400|2700
香蕉|2019年3月|13 | 2300|2700
### 2.8.1 行转列(列转行也支持)
```xml
<!-- 行转列 -->
<sql id="pivot_case">
<value>
<![CDATA[
select t.fruit_name,t.order_month,t.sale_count,t.sale_quantity,t.total_amt
from sqltoy_fruit_order t
order by t.fruit_name ,t.order_month
]]>
</value>
<!-- 行转列,将order_month作为分类横向标题,从sale_count列到total_amt 三个指标旋转成行 -->
<pivot start-column="sale_count" end-column="total_amt" group-columns="fruit_name" category-columns="order_month" />
</sql>
```
* 效果
<table>
<thead>
<tr>
<th rowspan="2">品类</th>
<th colspan="3">2019年3月</th>
<th colspan="3">2019年4月</th>
<th colspan="3">2019年5月</th>
</tr>
<tr>
<th>笔数</th><th>数量</th><th>总金额</th>
<th>笔数</th><th>数量</th><th>总金额</th>
<th>笔数</th><th>数量</th><th>总金额</th>
</tr>
</thead>
<tbody>
<tr>
<td>香蕉</td>
<td>13</td>
<td>2300</td>
<td>2700</td>
<td>12</td>
<td>2400</td>
<td>2700</td>
<td>10</td>
<td>2000</td>
<td>2000</td>
</tr>
<tr>
<td>苹果</td>
<td>13</td>
<td>2000</td>
<td>2500</td>
<td>11</td>
<td>1900</td>
<td>2600</td>
<td>12</td>
<td>2000</td>
<td>2400</td>
</tr>
</tbody>
</table>
### 2.8.2 分组汇总、求平均(可任意层级)
```xml
<sql id="group_summary_case">
<value>
<![CDATA[
select t.fruit_name,t.order_month,t.sale_count,t.sale_quantity,t.total_amt
from sqltoy_fruit_order t
order by t.fruit_name ,t.order_month
]]>
</value>
<!-- reverse 是否反向 -->
<summary columns="sale_count,sale_quantity,total_amt" reverse="true">
<!-- 层级顺序保持从高到低 -->
<global sum-label="总计" label-column="fruit_name" />
<!-- order-column: 分组排序列(对同分组进行排序),order-with-sum:默认为true,order-way:desc/asc -->
<group group-column="fruit_name" sum-label="小计" label-column="fruit_name" />
</summary>
</sql>
```
* 效果
品类|销售月份|销售笔数|销售数量(吨)|销售金额(万元)
----|-------|-------|----------|------------
总计| | 71 | 12600 |14900
小计| | 36 | 5900 | 7500
苹果|2019年5月|12 | 2000|2400
苹果|2019年4月|11 | 1900|2600
苹果|2019年3月|13 | 2000|2500
小计| | 35 | 6700|7400
香蕉|2019年5月|10 | 2000|2000
香蕉|2019年4月|12 | 2400|2700
香蕉|2019年3月|13 | 2300|2700
### 2.8.3 先行转列再环比计算
```xml
<!-- 列与列环比演示 -->
<sql id="cols_relative_case">
<value>
<![CDATA[
select t.fruit_name,t.order_month,t.sale_count,t.sale_amt,t.total_amt
from sqltoy_fruit_order t
order by t.fruit_name ,t.order_month
]]>
</value>
<!-- 数据旋转,行转列,将order_month 按列显示,每个月份下面有三个指标 -->
<pivot start-column="sale_count" end-column="total_amt" group-columns="fruit_name" category-columns="order_month" />
<!-- 列与列之间进行环比计算 -->
<cols-chain-relative group-size="3" relative-indexs="1,2" start-column="1" format="#.00%" />
</sql>
```
* 效果
<table>
<thead>
<tr>
<th rowspan="2" nowrap="nowrap">品类</th>
<th colspan="5">2019年3月</th>
<th colspan="5">2019年4月</th>
<th colspan="5">2019年5月</th>
</tr>
<tr>
<th nowrap="nowrap">笔数</th><th nowrap="nowrap">数量</th><th nowrap="nowrap">比上月</th><th nowrap="nowrap">总金额</th><th nowrap="nowrap">比上月</th>
<th nowrap="nowrap">笔数</th><th nowrap="nowrap">数量</th><th nowrap="nowrap">比上月</th><th nowrap="nowrap">总金额</th><th nowrap="nowrap">比上月</th>
<th nowrap="nowrap">笔数</th><th nowrap="nowrap">数量</th><th nowrap="nowrap">比上月</th><th nowrap="nowrap">总金额</th><th nowrap="nowrap">比上月</th>
</tr>
</thead>
<tbody>
<tr>
<td>香蕉</td>
<td>13</td>
<td>2300</td>
<td></td>
<td>2700</td>
<td></td>
<td>12</td>
<td>2400</td>
<td>4.30%</td>
<td>2700</td>
<td>0.00%</td>
<td>10</td>
<td>2000</td>
<td>-16.70%</td>
<td>2000</td>
<td>-26.00%</td>
</tr>
<tr>
<td>苹果</td>
<td>13</td>
<td>2000</td>
<td></td>
<td>2500</td>
<td></td>
<td>11</td>
<td>1900</td>
<td>-5.10%</td>
<td>2600</td>
<td>4.00%</td>
<td>12</td>
<td>2000</td>
<td>5.20%</td>
<td>2400</td>
<td>-7.70%</td>
</tr>
</tbody>
</table>
### 2.8.4 树排序汇总
```xml
<!-- 树排序、汇总 -->
<sql id="treeTable_sort_sum">
<value>
<![CDATA[
select t.area_code,t.pid_area,sale_cnt from sqltoy_area_sales t
]]>
</value>
<!-- 组织树形上下归属结构,同时将底层节点值逐层汇总到父节点上,并且对同层级按照降序排列 -->
<tree-sort id-column="area_code" pid-column="pid_area" sum-columns="sale_cnt" level-order-column="sale_cnt" order-way="desc"/>
</sql>
```
* 效果
<table>
<thead>
<tr>
<th>地区</th>
<th>归属地区</th>
<th>销售量</th>
</tr>
</thead>
<tbody>
<tr>
<td>上海</td>
<td>中国</td>
<td>300</td>
</tr>
<tr>
<td> 松江</td>
<td>上海</td>
<td> 120</td>
</tr>
<tr>
<td> 杨浦</td>
<td>上海</td>
<td> 116</td>
</tr>
<tr>
<td> 浦东</td>
<td>上海</td>
<td> 64</td>
</tr>
<tr>
<td>江苏</td>
<td>中国</td>
<td>270</td>
</tr>
<tr>
<td> 南京</td>
<td>江苏</td>
<td> 110</td>
</tr>
<tr>
<td> 苏州</td>
<td>江苏</td>
<td> 90</td>
</tr>
<tr>
<td> 无锡</td>
<td>江苏</td>
<td> 70</td>
</tr>
</tbody>
</table>
## 2.9 分库分表
### 2.9.1 查询分库分表(分库和分表策略可以同时使用)
```xml
sql参见quickstart项目:com/sqltoy/quickstart/sqltoy-quickstart.sql.xml 文件
<!-- 演示分库 -->
<sql id="qstart_db_sharding_case">
<sharding-datasource strategy="hashDataSource" params="userId" />
<value>
<![CDATA[
select * from sqltoy_user_log t
-- userId 作为分库关键字段属于必备条件
where t.user_id=:userId
#[and t.log_date>=:beginDate]
#[and t.log_date<=:endDate]
]]>
</value>
</sql>
<!-- 演示分表 -->
<sql id="qstart_sharding_table_case">
<sharding-table tables="sqltoy_trans_info_15d" strategy="realHisTable" params="beginDate" />
<value><![CDATA[
select * from sqltoy_trans_info_15d t
where t.trans_date>=:beginDate
#[and t.trans_date<=:endDate]
]]>
</value>
</sql>
```
### 2.9.2 操作分库分表(vo对象由quickvo工具自动根据数据库生成,且自定义的注解不会被覆盖)
@Sharding 在对象上通过注解来实现分库分表的策略配置
参见:com.sqltoy.quickstart.ShardingSearchTest 进行演示
```java
package com.sqltoy.showcase.vo;
import java.time.LocalDate;
import java.time.LocalDateTime;
import org.sagacity.sqltoy.config.annotation.Sharding;
import org.sagacity.sqltoy.config.annotation.SqlToyEntity;
import org.sagacity.sqltoy.config.annotation.Strategy;
import com.sagframe.sqltoy.showcase.vo.base.AbstractUserLogVO;
/**
* @project sqltoy-showcase
* @author zhongxuchen
* @version 1.0.0 Table: sqltoy_user_log,Remark:用户日志表
*/
/*
* db则是分库策略配置,table 则是分表策略配置,可以同时配置也可以独立配置
* 策略name要跟spring中的bean定义name一致,fields表示要以对象的哪几个字段值作为判断依据,可以一个或多个字段
* maxConcurrents:可选配置,表示最大并行数 maxWaitSeconds:可选配置,表示最大等待秒数
*/
@Sharding(db = @Strategy(name = "hashBalanceDBSharding", fields = { "userId" }),
// table = @Strategy(name = "hashBalanceSharding", fields = {"userId" }),
maxConcurrents = 10, maxWaitSeconds = 1800)
@SqlToyEntity
public class UserLogVO extends AbstractUserLogVO {
/**
*
*/
private static final long serialVersionUID = 1296922598783858512L;
/** default constructor */
public UserLogVO() {
super();
}
}
```
# 3.集成说明
## 3.1 参见trunk 下面的quickstart,并阅读readme.md进行上手
```java
package com.sqltoy.quickstart;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.transaction.annotation.EnableTransactionManagement;
/**
*
* @project sqltoy-quickstart
* @description quickstart 主程序入口
* @author zhongxuchen
* @version v1.0, Date:2020年7月17日
* @modify 2020年7月17日,修改说明
*/
@SpringBootApplication
@ComponentScan(basePackages = { "com.sqltoy.config", "com.sqltoy.quickstart" })
@EnableTransactionManagement
public class SqlToyApplication {
/**
* @param args
*/
public static void main(String[] args) {
SpringApplication.run(SqlToyApplication.class, args);
}
}
```
## 3.2 application.properties sqltoy部分配置
```properties
# sqltoy config
spring.sqltoy.sqlResourcesDir=classpath:com/sqltoy/quickstart
spring.sqltoy.translateConfig=classpath:sqltoy-translate.xml
spring.sqltoy.debug=true
#spring.sqltoy.reservedWords=status,sex_type
#dataSourceSelector: org.sagacity.sqltoy.plugins.datasource.impl.DefaultDataSourceSelector
#spring.sqltoy.defaultDataSource=dataSource
spring.sqltoy.unifyFieldsHandler=com.sqltoy.plugins.SqlToyUnifyFieldsHandler
#spring.sqltoy.printSqlTimeoutMillis=200000
```
## 3.3 缓存翻译的配置文件sqltoy-translate.xml
```xml
<?xml version="1.0" encoding="UTF-8"?>
<sagacity
xmlns="http://www.sagframe.com/schema/sqltoy-translate"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.sagframe.com/schema/sqltoy-translate http://www.sagframe.com/schema/sqltoy/sqltoy-translate.xsd">
<!-- 缓存有默认失效时间,默认为1小时,因此只有较为频繁的缓存才需要及时检测 -->
<cache-translates>
<!-- 基于sql直接查询的方式获取缓存 -->
<sql-translate cache="dictKeyName"
datasource="dataSource">
<sql>
<![CDATA[
select t.DICT_KEY,t.DICT_NAME,t.STATUS
from SQLTOY_DICT_DETAIL t
where t.DICT_TYPE=:dictType
order by t.SHOW_INDEX
]]>
</sql>
</sql-translate>
</cache-translates>
<!-- 缓存刷新检测,可以提供多个基于sql、service、rest服务检测 -->
<cache-update-checkers>
<!-- 增量更新,带有内部分类的查询结果第一列是分类 -->
<sql-increment-checker cache="dictKeyName"
check-frequency="15" has-inside-group="true" datasource="dataSource">
<sql><![CDATA[
--#not_debug#--
select t.DICT_TYPE,t.DICT_KEY,t.DICT_NAME,t.STATUS
from SQLTOY_DICT_DETAIL t
where t.UPDATE_TIME >=:lastUpdateTime
]]></sql>
</sql-increment-checker>
</cache-update-checkers>
</sagacity>
```
* 实际业务开发使用,直接利用SqlToyCRUDService 就可以进行常规的操作,避免简单的对象操作自己写service,
另外针对复杂逻辑则自己写service直接通过调用sqltoy提供的:LightDao 完成数据库交互操作!
```java
@RunWith(SpringRunner.class)
@SpringBootTest(classes = SqlToyApplication.class)
public class CrudCaseServiceTest {
@Autowired
private SqlToyCRUDService sqlToyCRUDService;
/**
* 创建一条员工记录
*/
@Test
public void saveStaffInfo() {
StaffInfoVO staffInfo = new StaffInfoVO();
staffInfo.setStaffId("S190715005");
staffInfo.setStaffCode("S190715005");
staffInfo.setStaffName("测试员工4");
staffInfo.setSexType("M");
staffInfo.setEmail("test3@aliyun.com");
staffInfo.setEntryDate(LocalDate.now());
staffInfo.setStatus(1);
staffInfo.setOrganId("C0001");
staffInfo.setPhoto(FileUtil.readAsBytes("classpath:/mock/staff_photo.jpg"));
staffInfo.setCountry("86");
sqlToyCRUDService.save(staffInfo);
}
}
```
# 4. sqltoy关键代码说明
## 4.1 sqltoy-orm 主要分以下几个部分:
- SqlToyDaoSupport:提供给开发者Dao继承的基本Dao,集成了所有对数据库操作的方法。
- LightDao:提供给开发者快捷使用的Dao,让开发者只关注写Service业务逻辑代码,在service中直接调用lightDao
- DialectFactory:数据库方言工厂类,sqltoy根据当前连接的方言调用不同数据库的实现封装。
- SqlToyContext:sqltoy上下文配置,是整个框架的核心配置和交换区,spring配置主要是配置sqltoyContext。
- EntityManager:封装于SqlToyContext,用于托管POJO对象,建立对象跟数据库表的关系。sqltoy通过SqlToyEntity注解扫描加载对象。
- ScriptLoader:sql配置文件加载解析器,封装于SqlToyContext中。sql文件严格按照*.sql.xml规则命名。
- TranslateManager:缓存翻译管理器,用于加载缓存翻译的xml配置文件和缓存实现类,sqltoy提供了接口并提供了默认基于ehcache的本地缓存实现,这样效率是最高的,而redis这种分布式缓存IO开销太大,缓存翻译是一个高频度的调用,一般会缓存注入员工、机构、数据字典、产品品类、地区等相对变化不频繁的稳定数据。
- ShardingStragety:分库分表策略管理器,4.x版本之后策略管理器并不需要显式定义,只有通过spring定义,sqltoy会在使用时动态管理。
## 4.2 快速阅读理解sqltoy:
- 从LightDao作为入口,了解sqltoy提供的所有功能
- SqlToyDaoSupport 是LightDao 具体功能实现。
- 从DialectFactory会进入不同数据库方言的实现入口。可以跟踪看到具体数据库的实现逻辑。你会看到oracle、mysql等分页、取随机记录、快速分页的封装等。
- EntityManager:你会找到如何扫描POJO并构造成模型,知道通过POJO操作数据库实质会变成相应的sql进行交互。
- ParallelUtils:对象分库分表并行执行器,通过这个类你会看到分库分表批量操作时如何将集合分组到不同的库不同的表并进行并行调度的。
- SqlToyContext:sqltoy配置的上下文,通过这个类可以看到sqltoy全貌。
- PageOptimizeUtils:可以看到分页优化默认实现原理。
| 0 |
heibaiying/BigData-Notes | 大数据入门指南 :star: | azkaban big-data bigdata flume hadoop hbase hdfs hive kafka mapreduce phoenix scala spark sqoop storm yarn zookeeper | # BigData-Notes
<div align="center"> <img width="444px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/bigdata-notes-icon.png"/> </div>
<br/>
**大数据入门指南**
<table>
<tr>
<th><img width="50px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/hadoop.jpg"></th>
<th><img width="50px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/hive.jpg"></th>
<th><img width="50px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/spark.jpg"></th>
<th><img width="50px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/storm.png"></th>
<th><img width="50px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/flink.png"></th>
<th><img width="50px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/hbase.png"></th>
<th><img width="50px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/kafka.png"></th>
<th><img width="50px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/zookeeper.jpg"></th>
<th><img width="50px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/flume.png"></th>
<th><img width="50px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/sqoop.png"></th>
<th><img width="50px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/azkaban.png"></th>
<th><img width="50px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/scala.jpg"></th>
</tr>
<tr>
<td align="center"><a href="#一hadoop">Hadoop</a></td>
<td align="center"><a href="#二hive">Hive</a></td>
<td align="center"><a href="#三spark">Spark</a></td>
<td align="center"><a href="#四storm">Storm</a></td>
<td align="center"><a href="#五flink">Flink</a></td>
<td align="center"><a href="#六hbase">HBase</a></td>
<td align="center"><a href="#七kafka">Kafka</a></td>
<td align="center"><a href="#八zookeeper">Zookeeper</a></td>
<td align="center"><a href="#九flume">Flume</a></td>
<td align="center"><a href="#十sqoop">Sqoop</a></td>
<td align="center"><a href="#十一azkaban">Azkaban</a></td>
<td align="center"><a href="#十二scala">Scala</a></td>
</tr>
</table>
<br/>
<div align="center">
<a href = "https://github.com/heibaiying/Full-Stack-Notes">
<img width="150px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/weixin.jpg"/>
</a>
</div>
<div align="center"> <strong> 如果需要离线阅读,可以在公众号上发送 “bigdata” 获取《大数据入门指南》离线阅读版! </strong> </div>
<br/>
## :black_nib: 前 言
1. [大数据学习路线](notes/大数据学习路线.md)
2. [大数据技术栈思维导图](notes/大数据技术栈思维导图.md)
3. [大数据常用软件安装指南](notes/大数据常用软件安装指南.md)
## 一、Hadoop
1. [分布式文件存储系统 —— HDFS](notes/Hadoop-HDFS.md)
2. [分布式计算框架 —— MapReduce](notes/Hadoop-MapReduce.md)
3. [集群资源管理器 —— YARN](notes/Hadoop-YARN.md)
4. [Hadoop 单机伪集群环境搭建](notes/installation/Hadoop单机环境搭建.md)
5. [Hadoop 集群环境搭建](notes/installation/Hadoop集群环境搭建.md)
6. [HDFS 常用 Shell 命令](notes/HDFS常用Shell命令.md)
7. [HDFS Java API 的使用](notes/HDFS-Java-API.md)
8. [基于 Zookeeper 搭建 Hadoop 高可用集群](notes/installation/基于Zookeeper搭建Hadoop高可用集群.md)
## 二、Hive
1. [Hive 简介及核心概念](notes/Hive简介及核心概念.md)
2. [Linux 环境下 Hive 的安装部署](notes/installation/Linux环境下Hive的安装部署.md)
4. [Hive CLI 和 Beeline 命令行的基本使用](notes/HiveCLI和Beeline命令行的基本使用.md)
6. [Hive 常用 DDL 操作](notes/Hive常用DDL操作.md)
7. [Hive 分区表和分桶表](notes/Hive分区表和分桶表.md)
8. [Hive 视图和索引](notes/Hive视图和索引.md)
9. [Hive 常用 DML 操作](notes/Hive常用DML操作.md)
10. [Hive 数据查询详解](notes/Hive数据查询详解.md)
## 三、Spark
**Spark Core :**
1. [Spark 简介](notes/Spark简介.md)
2. [Spark 开发环境搭建](notes/installation/Spark开发环境搭建.md)
4. [弹性式数据集 RDD](notes/Spark_RDD.md)
5. [RDD 常用算子详解](notes/Spark_Transformation和Action算子.md)
5. [Spark 运行模式与作业提交](notes/Spark部署模式与作业提交.md)
6. [Spark 累加器与广播变量](notes/Spark累加器与广播变量.md)
7. [基于 Zookeeper 搭建 Spark 高可用集群](notes/installation/Spark集群环境搭建.md)
**Spark SQL :**
1. [DateFrame 和 DataSet ](notes/SparkSQL_Dataset和DataFrame简介.md)
2. [Structured API 的基本使用](notes/Spark_Structured_API的基本使用.md)
3. [Spark SQL 外部数据源](notes/SparkSQL外部数据源.md)
4. [Spark SQL 常用聚合函数](notes/SparkSQL常用聚合函数.md)
5. [Spark SQL JOIN 操作](notes/SparkSQL联结操作.md)
**Spark Streaming :**
1. [Spark Streaming 简介](notes/Spark_Streaming与流处理.md)
2. [Spark Streaming 基本操作](notes/Spark_Streaming基本操作.md)
3. [Spark Streaming 整合 Flume](notes/Spark_Streaming整合Flume.md)
4. [Spark Streaming 整合 Kafka](notes/Spark_Streaming整合Kafka.md)
## 四、Storm
1. [Storm 和流处理简介](notes/Storm和流处理简介.md)
2. [Storm 核心概念详解](notes/Storm核心概念详解.md)
3. [Storm 单机环境搭建](notes/installation/Storm单机环境搭建.md)
4. [Storm 集群环境搭建](notes/installation/Storm集群环境搭建.md)
5. [Storm 编程模型详解](notes/Storm编程模型详解.md)
6. [Storm 项目三种打包方式对比分析](notes/Storm三种打包方式对比分析.md)
7. [Storm 集成 Redis 详解](notes/Storm集成Redis详解.md)
8. [Storm 集成 HDFS/HBase](notes/Storm集成HBase和HDFS.md)
9. [Storm 集成 Kafka](notes/Storm集成Kakfa.md)
## 五、Flink
1. [Flink 核心概念综述](notes/Flink核心概念综述.md)
2. [Flink 开发环境搭建](notes/Flink开发环境搭建.md)
3. [Flink Data Source](notes/Flink_Data_Source.md)
4. [Flink Data Transformation](notes/Flink_Data_Transformation.md)
4. [Flink Data Sink](notes/Flink_Data_Sink.md)
6. [Flink 窗口模型](notes/Flink_Windows.md)
7. [Flink 状态管理与检查点机制](notes/Flink状态管理与检查点机制.md)
8. [Flink Standalone 集群部署](notes/installation/Flink_Standalone_Cluster.md)
## 六、HBase
1. [Hbase 简介](notes/Hbase简介.md)
2. [HBase 系统架构及数据结构](notes/Hbase系统架构及数据结构.md)
3. [HBase 基本环境搭建 (Standalone /pseudo-distributed mode)](notes/installation/HBase单机环境搭建.md)
4. [HBase 集群环境搭建](notes/installation/HBase集群环境搭建.md)
5. [HBase 常用 Shell 命令](notes/Hbase_Shell.md)
6. [HBase Java API](notes/Hbase_Java_API.md)
7. [HBase 过滤器详解](notes/Hbase过滤器详解.md)
8. [HBase 协处理器详解](notes/Hbase协处理器详解.md)
9. [HBase 容灾与备份](notes/Hbase容灾与备份.md)
10. [HBase的 SQL 中间层 —— Phoenix](notes/Hbase的SQL中间层_Phoenix.md)
11. [Spring/Spring Boot 整合 Mybatis + Phoenix](notes/Spring+Mybtais+Phoenix整合.md)
## 七、Kafka
1. [Kafka 简介](notes/Kafka简介.md)
2. [基于 Zookeeper 搭建 Kafka 高可用集群](notes/installation/基于Zookeeper搭建Kafka高可用集群.md)
3. [Kafka 生产者详解](notes/Kafka生产者详解.md)
4. [Kafka 消费者详解](notes/Kafka消费者详解.md)
5. [深入理解 Kafka 副本机制](notes/Kafka深入理解分区副本机制.md)
## 八、Zookeeper
1. [Zookeeper 简介及核心概念](notes/Zookeeper简介及核心概念.md)
2. [Zookeeper 单机环境和集群环境搭建](notes/installation/Zookeeper单机环境和集群环境搭建.md)
3. [Zookeeper 常用 Shell 命令](notes/Zookeeper常用Shell命令.md)
4. [Zookeeper Java 客户端 —— Apache Curator](notes/Zookeeper_Java客户端Curator.md)
5. [Zookeeper ACL 权限控制](notes/Zookeeper_ACL权限控制.md)
## 九、Flume
1. [Flume 简介及基本使用](notes/Flume简介及基本使用.md)
2. [Linux 环境下 Flume 的安装部署](notes/installation/Linux下Flume的安装.md)
3. [Flume 整合 Kafka](notes/Flume整合Kafka.md)
## 十、Sqoop
1. [Sqoop 简介与安装](notes/Sqoop简介与安装.md)
2. [Sqoop 的基本使用](notes/Sqoop基本使用.md)
## 十一、Azkaban
1. [Azkaban 简介](notes/Azkaban简介.md)
2. [Azkaban3.x 编译及部署](notes/installation/Azkaban_3.x_编译及部署.md)
3. [Azkaban Flow 1.0 的使用](notes/Azkaban_Flow_1.0_的使用.md)
4. [Azkaban Flow 2.0 的使用](notes/Azkaban_Flow_2.0_的使用.md)
## 十二、Scala
1. [Scala 简介及开发环境配置](notes/Scala简介及开发环境配置.md)
2. [基本数据类型和运算符](notes/Scala基本数据类型和运算符.md)
3. [流程控制语句](notes/Scala流程控制语句.md)
4. [数组 —— Array](notes/Scala数组.md)
5. [集合类型综述](notes/Scala集合类型.md)
6. [常用集合类型之 —— List & Set](notes/Scala列表和集.md)
7. [常用集合类型之 —— Map & Tuple](notes/Scala映射和元组.md)
8. [类和对象](notes/Scala类和对象.md)
9. [继承和特质](notes/Scala继承和特质.md)
10. [函数 & 闭包 & 柯里化](notes/Scala函数和闭包.md)
11. [模式匹配](notes/Scala模式匹配.md)
12. [类型参数](notes/Scala类型参数.md)
13. [隐式转换和隐式参数](notes/Scala隐式转换和隐式参数.md)
## 十三、公共内容
1. [大数据应用常用打包方式](notes/大数据应用常用打包方式.md)
<br>
## :bookmark_tabs: 后 记
[资料分享与开发工具推荐](notes/资料分享与工具推荐.md)
<br>
<div align="center">
<a href = "https://blog.csdn.net/m0_37809146">
<img width="200px" src="https://gitee.com/heibaiying/BigData-Notes/raw/master/pictures/blog-logo.png"/>
</a>
</div>
<div align="center"> <a href = "https://blog.csdn.net/m0_37809146"> 欢迎关注我的博客:https://blog.csdn.net/m0_37809146</a> </div>
| 0 |
spring-io/initializr | A quickstart generator for Spring projects | null | null | 0 |
core-lib/xjar | Spring Boot JAR 安全加密运行工具,支持的原生JAR。 | aes classloader decompilation decryption encryption jar springboot | # XJar [](https://jitpack.io/#core-lib/xjar)
GitHub: https://github.com/core-lib/xjar
### Spring Boot JAR 安全加密运行工具, 同时支持的原生JAR.
### 基于对JAR包内资源的加密以及拓展ClassLoader来构建的一套程序加密启动, 动态解密运行的方案, 避免源码泄露以及反编译.
## 功能特性
* 无代码侵入, 只需要把编译好的JAR包通过工具加密即可.
* 完全内存解密, 降低源码以及字节码泄露或反编译的风险.
* 支持所有JDK内置加解密算法.
* 可选择需要加解密的字节码或其他资源文件.
* 支持Maven插件, 加密更加便捷.
* 动态生成Go启动器, 保护密码不泄露.
## 环境依赖
JDK 1.7 +
## 使用步骤
#### 1. 添加依赖
```xml
<project>
<!-- 设置 jitpack.io 仓库 -->
<repositories>
<repository>
<id>jitpack.io</id>
<url>https://jitpack.io</url>
</repository>
</repositories>
<!-- 添加 XJar 依赖 -->
<dependencies>
<dependency>
<groupId>com.github.core-lib</groupId>
<artifactId>xjar</artifactId>
<version>4.0.2</version>
<!-- <scope>test</scope> -->
</dependency>
</dependencies>
</project>
```
* 必须添加 https://jitpack.io Maven仓库.
* 如果使用 JUnit 测试类来运行加密可以将 XJar 依赖的 scope 设置为 test.
#### 2. 加密源码
```java
XCryptos.encryption()
.from("/path/to/read/plaintext.jar")
.use("io.xjar")
.include("/io/xjar/**/*.class")
.include("/mapper/**/*Mapper.xml")
.exclude("/static/**/*")
.exclude("/conf/*")
.to("/path/to/save/encrypted.jar");
```
<table>
<thead>
<tr>
<th>方法名称</th><th>参数列表</th><th>是否必选</th><th>方法说明</th>
</tr>
</thead>
<tbody>
<tr>
<td>from</td><td>(String jar)</td><td rowspan="2">二选一</td><td>指定待加密JAR包路径</td>
</tr>
<tr>
<td>from</td><td>(File jar)</td><td>指定待加密JAR包文件</td>
</tr>
<tr>
<td>use</td><td>(String password)</td><td rowspan="2">二选一</td><td>指定加密密码</td>
</tr>
<tr>
<td>use</td><td>(String algorithm, int keysize, int ivsize, String password)</td><td>指定加密算法及加密密码</td>
</tr>
<tr>
<td>include</td><td>(String ant)</td><td>可多次调用</td><td>指定要加密的资源相对于classpath的ANT路径表达式</td>
</tr>
<tr>
<td>include</td><td>(Pattern regex)</td><td>可多次调用</td><td>指定要加密的资源相对于classpath的正则路径表达式</td>
</tr>
<tr>
<td>exclude</td><td>(String ant)</td><td>可多次调用</td><td>指定不加密的资源相对于classpath的ANT路径表达式</td>
</tr>
<tr>
<td>exclude</td><td>(Pattern regex)</td><td>可多次调用</td><td>指定不加密的资源相对于classpath的正则路径表达式</td>
</tr>
<tr>
<td>to</td><td>(String xJar)</td><td rowspan="2">二选一</td><td>指定加密后JAR包输出路径, 并执行加密.</td>
</tr>
<tr>
<td>to</td><td>(File xJar)</td><td>指定加密后JAR包输出文件, 并执行加密.</td>
</tr>
</tbody>
</table>
* 指定加密算法的时候密钥长度以及向量长度必须在算法可支持范围内, 具体加密算法的密钥及向量长度请自行百度或谷歌.
* include 和 exclude 同时使用时即加密在include的范围内且排除了exclude的资源.
#### 3. 编译脚本
```shell script
go build xjar.go
```
* 通过步骤2加密成功后XJar会在输出的JAR包同目录下生成一个名为 xjar.go 的的Go启动器源码文件.
* 将 xjar.go 在不同的平台进行编译即可得到不同平台的启动器可执行文件, 其中Windows下文件名为 xjar.exe 而Linux下为 xjar.
* 用于编译的机器需要安装 Go 环境, 用于运行的机器则可不必安装 Go 环境, 具体安装教程请自行搜索.
* 由于启动器自带JAR包防篡改校验, 故启动器无法通用, 即便密码相同也不行.
#### 4. 启动运行
```shell script
/path/to/xjar /path/to/java [OPTIONS] -jar /path/to/encrypted.jar [ARGS]
/path/to/xjar /path/to/javaw [OPTIONS] -jar /path/to/encrypted.jar [ARGS]
nohup /path/to/xjar /path/to/java [OPTIONS] -jar /path/to/encrypted.jar [ARGS]
```
* 在 Java 启动命令前加上编译好的Go启动器可执行文件名(xjar)即可启动运行加密后的JAR包.
* 若使用 nohup 方式启动则 nohup 要放在Go启动器可执行文件名(xjar)之前.
* 若Go启动器可执行文件名(xjar)不在当前命令行所在目录则要通过绝对路径或相对路径指定.
* 仅支持通过 -jar 方式启动, 不支持-cp或-classpath的方式.
* -jar 后面必须紧跟着启动的加密jar文件路径
* 例子: 如果当前命令行就在 xjar 所在目录, java 环境变量也设置好了 ./xjar java -Xms256m -Xmx1024m -jar /path/to/encrypted.jar
## 注意事项
#### 1. 不兼容 spring-boot-maven-plugin 的 executable = true 以及 embeddedLaunchScript
```xml
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<!-- 需要将executable和embeddedLaunchScript参数删除, 目前还不能支持对该模式Jar的加密!后面可能会支持该方式的打包.
<configuration>
<executable>true</executable>
<embeddedLaunchScript>...</embeddedLaunchScript>
</configuration>
-->
</plugin>
```
#### 2. Spring Boot + JPA(Hibernate) 启动报错问题
如果项目中使用了 JPA 且实现为Hibernate时, 由于Hibernate自己解析加密后的Jar文件, 所以无法正常启动, 可以采用以下解决方案:
1. clone [XJar-Agent-Hibernate](https://github.com/core-lib/xjar-agent-hibernate) , 使用 mvn clean package 编译出 xjar-agent-hibernate-${version}.jar 文件
2. 采用 xjar java -javaagent:xjar-agent-hibernate-${version}.jar -jar your-spring-boot-app.jar 命令启动
#### 3. 静态文件浏览器无法加载完成问题
由于静态文件被加密后文件体积变大, Spring Boot 会采用文件的大小作为 Content-Length 头返回给浏览器,
但实际上通过 XJar 加载解密后文件大小恢复了原本的大小, 所以浏览器认为还没接收完导致一直等待服务端.
由此我们需要在加密时忽略静态文件的加密, 实际上静态文件也没加密的必要, 因为即便加密了用户在浏览器
查看源代码也是能看到完整的源码.通常情况下静态文件都会放在 static/ 和 META-INF/resources/ 目录下,
我们只需要在加密时通过 exclude 方法排除这些资源即可, 可以参考以下例子:
```java
XCryptos.encryption()
.from("/path/to/read/plaintext.jar")
.use("io.xjar")
.exclude("/static/**/*")
.exclude("/META-INF/resources/**/*")
.to("/path/to/save/encrypted.jar");
```
#### 4. JDK-9及以上版本由于模块化导致XJar无法使用 jdk.internal.loader 包的问题解决方案
在启动时添加参数 --add-opens java.base/jdk.internal.loader=ALL-UNNAMED
```shell script
xjar java --add-opens java.base/jdk.internal.loader=ALL-UNNAMED -jar /path/to/encrypted.jar
```
#### 5. 由于使用了阿里云Maven镜像导致无法从 jitpack.io 下载 XJar 依赖的问题
参考如下设置, 在镜像配置的 mirrorOf 元素中加入 ,!jitpack.io 结尾.
```xml
<mirror>
<id>alimaven</id>
<mirrorOf>central,!jitpack.io</mirrorOf>
<name>aliyun maven</name>
<url>http://maven.aliyun.com/nexus/content/repositories/central/</url>
</mirror>
```
## 插件集成
#### Maven项目可通过集成 [xjar-maven-plugin](https://github.com/core-lib/xjar-maven-plugin) 以免去每次加密都要执行一次上述的代码, 随着Maven构建自动生成加密后的JAR和Go启动器源码文件.
[xjar-maven-plugin](https://github.com/core-lib/xjar-maven-plugin) GitHub: https://github.com/core-lib/xjar-maven-plugin
```xml
<project>
<!-- 设置 jitpack.io 插件仓库 -->
<pluginRepositories>
<pluginRepository>
<id>jitpack.io</id>
<url>https://jitpack.io</url>
</pluginRepository>
</pluginRepositories>
<!-- 添加 XJar Maven 插件 -->
<build>
<plugins>
<plugin>
<groupId>com.github.core-lib</groupId>
<artifactId>xjar-maven-plugin</artifactId>
<version>4.0.2</version>
<executions>
<execution>
<goals>
<goal>build</goal>
</goals>
<phase>package</phase>
<!-- 或使用
<phase>install</phase>
-->
<configuration>
<password>io.xjar</password>
<!-- optional
<algorithm/>
<keySize/>
<ivSize/>
<includes>
<include/>
</includes>
<excludes>
<exclude/>
</excludes>
<sourceDir/>
<sourceJar/>
<targetDir/>
<targetJar/>
-->
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
```
#### 对于Spring Boot 项目或模块, 该插件要后于 spring-boot-maven-plugin 插件执行, 有两种方式:
* 将插件放置于 spring-boot-maven-plugin 的后面, 因为其插件的默认 phase 也是 package
* 将插件的 phase 设置为 install(默认值为:package), 打包命令采用 mvn clean install
#### 也可以通过Maven命令执行
```text
mvn xjar:build -Dxjar.password=io.xjar
mvn xjar:build -Dxjar.password=io.xjar -Dxjar.targetDir=/directory/to/save/target.xjar
```
#### 但通常情况下是让XJar插件绑定到指定的phase中自动执行, 这样就能在项目构建的时候自动构建出加密的包.
```text
mvn clean package -Dxjar.password=io.xjar
mvn clean install -Dxjar.password=io.xjar -Dxjar.targetDir=/directory/to/save/target.xjar
```
## 强烈建议
强烈建议不要在 pom.xml 的 xjar-maven-plugin 配置中写上密码,这样会导致打包出来的 xjar 包中的 pom.xml 文件保留着密码,极其容易暴露密码!强烈推荐通过 mvn 命令来指定加密密钥!
## 参数说明
| 参数名称 | 命令参数名称 | 参数说明 | 参数类型 | 缺省值 | 示例值 |
| :------ | :----------- | :------ | :------ | :----- | :----- |
| password | -Dxjar.password | 密码字符串 | String | 必须 | 任意字符串, io.xjar |
| algorithm | -Dxjar.algorithm | 加密算法名称 | String | AES/CBC/PKCS5Padding | JDK内置加密算法, 如:AES/CBC/PKCS5Padding 和 DES/CBC/PKCS5Padding |
| keySize | -Dxjar.keySize | 密钥长度 | int | 128 | 根据加密算法而定, 56, 128, 256 |
| ivSize | -Dxjar.ivSize | 密钥向量长度 | int | 128 | 根据加密算法而定, 128 |
| sourceDir | -Dxjar.sourceDir | 源jar所在目录 | File | ${project.build.directory} | 文件目录 |
| sourceJar | -Dxjar.sourceJar | 源jar名称 | String | ${project.build.finalName}.jar | 文件名称 |
| targetDir | -Dxjar.targetDir | 目标jar存放目录 | File | ${project.build.directory} | 文件目录 |
| targetJar | -Dxjar.targetJar | 目标jar名称 | String | ${project.build.finalName}.xjar | 文件名称 |
| includes | -Dxjar.includes | 需要加密的资源路径表达式 | String[] | 无 | io/xjar/** , mapper/*Mapper.xml , 支持Ant表达式 |
| excludes | -Dxjar.excludes | 无需加密的资源路径表达式 | String[] | 无 | static/** , META-INF/resources/** , 支持Ant表达式 |
* 指定加密算法的时候密钥长度以及向量长度必须在算法可支持范围内, 具体加密算法的密钥及向量长度请自行百度或谷歌.
* 当 includes 和 excludes 同时使用时即加密在includes的范围内且排除了excludes的资源.
更多文档:[xjar-maven-plugin](https://github.com/core-lib/xjar-maven-plugin)
## 版本记录
* 4.0.2
1. 安全性升级
* 4.0.1
1. 兼容JDK-9及以上版本
* 4.0.0
1. 加解密支持填充模式
2. 加解密支持IV-Parameter
3. 升级启动器
4. 移除危险模式
5. 拼写错误修正
6. 提供智能加密/解密器 避免使用失误
7. 删除多余的加密/解密方法
8. 修复有安全校验的nested-lib在不加密其内部资源情况下启动时也无法通过校验的问题
9. 去除命令启动和手输密码启动的方式只保留Go启动器的模式
10. 增加可读性更强的Fluent风格的加密/解密API
* 2.0.9
1. 修复XJar类加载器加载的类没有 ProtectionDomain 以及 CodeSource 的问题
2. 去除版本号前置的"v"
* v2.0.7
1. 修复不同字符集机器间加密与运行的问题
* v2.0.6
1. 解决多jar包启动时无法找到准确的MANIFEST.MF导致无法正常启动的问题
* v2.0.5
1. 升级[LoadKit](https://github.com/core-lib/loadkit)依赖版本
2. 修复ANT表达式无法正确匹配**/*通配符的问题
* v2.0.4
1. 解决危险模式不支持ubuntu系统的问题
* v2.0.3
1. 过滤器泛型协变支持
2. xjar-maven-plugin 支持 includes 与 excludes 同时起效, 当同时设置时即加密在includes范围内但又不在excludes范围内的资源
* v2.0.2
1. 原生jar增加密钥文件的启动方式, 解决类似 nohup 和 javaw 的后台启动方式无法通过控制台输入密码的问题
* v2.0.1
1. 增加密钥文件的启动方式, 解决类似 nohup 和 javaw 的后台启动方式无法通过控制台输入密码的问题
2. 修复解密后没有删除危险模式中在MANIFEST.MF中保留的密钥信息
* v2.0.0
1. 支持内嵌JAR包资源的过滤加解密
2. 不兼容v1.x.x的过滤器表达式, 统一采用相对于 classpath 资源URL的过滤表达式
* v1.1.4
1. 支持 Spring-Boot 以ZIP方式打包, 即依赖外部化方式启动.
2. 修复无加密资源时无法启动问题
* v1.1.3
1. 实现危险模式加密启动, 即不需要输入密码!
2. 修复无法使用 System.console(); 时用 new Scanner(System.in) 替代.
* v1.1.2
1. 避免用户由于过滤器使用不当造成无法启动的风险
* v1.1.1
1. 修复bug
* v1.1.0
1. 整理目录结构
2. 增加正则表达式/Ant表达式过滤器和“非”(!)逻辑运算过滤器
3. 将XEntryFilters工具类整合在XKit类中
4. 缺省过滤器情况下Spring-Boot JAR包加密的资源只限定在 BOOT-INF/classes/ 下
* v1.0.9
1. 修复对Spring-Boot 版本依赖的bug
* v1.0.8
1. 支持以Maven插件方式集成
* v1.0.7
1. 将sprint-boot-loader依赖设为provide
2. 将XEntryFilter#filter(E entry); 变更为XEntryFilter#filtrate(E entry);
3. 将Encryptor/Decryptor的构造函数中接收多个过滤器参数变成接收一个, 外部提供XEntryFilters工具类来实现多过滤器混合成一个, 避免框架自身的逻辑限制了使用者的过滤逻辑实现.
* v1.0.6
1. 采用[LoadKit](https://github.com/core-lib/loadkit)作为资源加载工具
* v1.0.5
1. 支持并行类加载, 需要JDK1.7+的支持, 可提升多线程环境类加载的效率
2. Spring-Boot JAR 包加解密增加一个安全过滤器, 避免无关资源被加密造成无法运行
3. XBoot / XJar 工具类中增加多个按文件路径加解密的方法, 提升使用便捷性
* v1.0.4 小优化
* v1.0.3 增加Spring-Boot的FatJar加解密时的缺省过滤器, 避免由于没有提供过滤器时加密后的JAR包不能正常运行.
* v1.0.2 修复中文及空格路径的问题
* v1.0.1 升级detector框架
* v1.0.0 第一个正式版发布
## 协议声明
[Apache-2.0](http://www.apache.org/licenses/LICENSE-2.0)
## 加入群聊
QQ 950956093 [](https://shang.qq.com/wpa/qunwpa?idkey=e567db1c32de4b02da480d895566757b3df73e3f8827ed6c9149e2859e4cdc93)
| 0 |
alibaba/nacos | an easy-to-use dynamic service discovery, configuration and service management platform for building cloud native applications. | alibaba config configuration-management distributed-configuration dns dubbo istio kubernetes microservices nacos service-discovery service-mesh spring-cloud |
<img src="doc/Nacos_Logo.png" width="50%" syt height="50%" />
# Nacos: Dynamic *Na*ming and *Co*nfiguration *S*ervice
[](https://gitter.im/alibaba/nacos?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge) [](https://www.apache.org/licenses/LICENSE-2.0.html)
[](https://travis-ci.org/alibaba/nacos)
[](https://opensource.alibaba.com/contribution_leaderboard/details?projectValue=nacos)
-------
## What does it do
Nacos (official site: [nacos.io](https://nacos.io)) is an easy-to-use platform designed for dynamic service discovery and configuration and service management. It helps you to build cloud native applications and microservices platform easily.
Service is a first-class citizen in Nacos. Nacos supports almost all type of services,for example,[Dubbo/gRPC service](https://nacos.io/en-us/docs/use-nacos-with-dubbo.html), [Spring Cloud RESTFul service](https://nacos.io/en-us/docs/use-nacos-with-springcloud.html) or [Kubernetes service](https://nacos.io/en-us/docs/use-nacos-with-kubernetes.html).
Nacos provides four major functions.
* **Service Discovery and Service Health Check**
Nacos makes it simple for services to register themselves and to discover other services via a DNS or HTTP interface. Nacos also provides real-time health checks of services to prevent sending requests to unhealthy hosts or service instances.
* **Dynamic Configuration Management**
Dynamic Configuration Service allows you to manage configurations of all services in a centralized and dynamic manner across all environments. Nacos eliminates the need to redeploy applications and services when configurations are updated, which makes configuration changes more efficient and agile.
* **Dynamic DNS Service**
Nacos supports weighted routing, making it easier for you to implement mid-tier load balancing, flexible routing policies, flow control, and simple DNS resolution services in the production environment within your data center. It helps you to implement DNS-based service discovery easily and prevent applications from coupling to vendor-specific service discovery APIs.
* **Service and MetaData Management**
Nacos provides an easy-to-use service dashboard to help you manage your services metadata, configuration, kubernetes DNS, service health and metrics statistics.
## Quick Start
It is super easy to get started with your first project.
### Deploying Nacos on cloud
You can deploy Nacos on cloud, which is the easiest and most convenient way to start Nacos.
Use the following [Nacos deployment guide](https://cn.aliyun.com/product/aliware/mse?spm=nacos-website.topbar.0.0.0) to see more information and deploy a stable and out-of-the-box Nacos server.
### Start by the provided startup package
#### Step 1: Download the binary package
You can download the package from the [latest stable release](https://github.com/alibaba/nacos/releases).
Take release `nacos-server-1.0.0.zip` for example:
```sh
unzip nacos-server-1.0.0.zip
cd nacos/bin
```
#### Step 2: Start Server
On the **Linux/Unix/Mac** platform, run the following command to start server with standalone mode:
```sh
sh startup.sh -m standalone
```
On the **Windows** platform, run the following command to start server with standalone mode. Alternatively, you can also double-click the `startup.cmd` to run NacosServer.
```
startup.cmd -m standalone
```
For more details, see [quick-start.](https://nacos.io/en-us/docs/quick-start.html)
## Quick start for other open-source projects:
* [Quick start with Nacos command and console](https://nacos.io/en-us/docs/quick-start.html)
* [Quick start with dubbo](https://nacos.io/en-us/docs/use-nacos-with-dubbo.html)
* [Quick start with spring cloud](https://nacos.io/en-us/docs/quick-start-spring-cloud.html)
* [Quick start with kubernetes](https://nacos.io/en-us/docs/use-nacos-with-kubernetes.html)
## Documentation
You can view the full documentation from the [Nacos website](https://nacos.io/en-us/docs/v2/what-is-nacos.html).
You can also read this online eBook from the [NACOS ARCHITECTURE & PRINCIPLES](https://www.yuque.com/nacos/ebook/kbyo6n).
All the latest and long-term notice can also be found here from [Github notice issue](https://github.com/alibaba/nacos/labels/notice).
## Contributing
Contributors are welcomed to join Nacos project. Please check [CONTRIBUTING](./CONTRIBUTING.md) about how to contribute to this project.
### How can I contribute?
* Take a look at issues with tags marked [`good first issue`](https://github.com/alibaba/nacos/issues?q=is%3Aopen+is%3Aissue+label%3A%22good+first+issue%22) or [`contribution welcome`](https://github.com/alibaba/nacos/issues?q=is%3Aopen+is%3Aissue+label%3A%22contribution+welcome%22).
* Answer questions on [issues](https://github.com/alibaba/nacos/issues).
* Fix bugs reported on [issues](https://github.com/alibaba/nacos/issues), and send us a pull request.
* Review the existing [pull request](https://github.com/alibaba/nacos/pulls).
* Improve the [website](https://github.com/nacos-group/nacos-group.github.io), typically we need
* blog post
* translation on documentation
* use cases around the integration of Nacos in enterprise systems.
## Other Related Project Repositories
* [nacos-spring-project](https://github.com/nacos-group/nacos-spring-project) provides the integration functionality for Spring.
* [nacos-group](https://github.com/nacos-group) is the repository that hosts the eco tools for Nacos, such as SDK, synchronization tool, etc.
* [spring-cloud-alibaba](https://github.com/spring-cloud-incubator/spring-cloud-alibaba) provides the one-stop solution for application development over Alibaba middleware which includes Nacos.
## Contact
* [Gitter](https://gitter.im/alibaba/nacos): Nacos's IM tool for community messaging, collaboration and discovery.
* [Twitter](https://twitter.com/nacos2): Follow along for latest nacos news on Twitter.
* [Weibo](https://weibo.com/u/6574374908): Follow along for latest nacos news on Weibo (Twitter of China version).
* [Nacos Segmentfault](https://segmentfault.com/t/nacos): Get latest notice and prompt help from Segmentfault.
* Email Group:
* users-nacos@googlegroups.com: Nacos usage general discussion.
* dev-nacos@googlegroups.com: Nacos developer discussion (APIs, feature design, etc).
* commits-nacos@googlegroups.com: Commits notice, very high frequency.
* Join us from DingDing(Group 1: 21708933(full), Group 2: 30438813(full), Group 3: 31222241(full), Group 4: 12810027056).
<img src="https://img.alicdn.com/imgextra/i4/O1CN01MI7re41xTrZNdB7Yv_!!6000000006445-0-tps-974-1228.jpg" width="500">
## Enterprise Service
If you need Nacos enterprise service support, or purchase cloud product services, you can join the discussion by scanning the following DingTalk group. It can also be directly activated and used through the microservice engine (MSE) provided by Alibaba Cloud.
https://cn.aliyun.com/product/aliware/mse?spm=nacos-website.topbar.0.0.0
<img src="https://img.alicdn.com/imgextra/i3/O1CN01RTfN7q1KUzX4TcH08_!!6000000001168-2-tps-864-814.png" width="500">
## Download
- [Nacos Official Website](https://nacos.io/download/nacos-server)
- [Github Release](https://github.com/alibaba/nacos/releases)
## Who is using
These are only part of the companies using Nacos, for reference only. If you are using Nacos, please [add your company here](https://github.com/alibaba/nacos/issues/273) to tell us your scenario to make Nacos better.

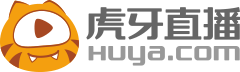

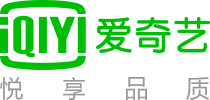

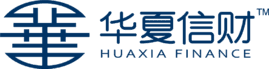
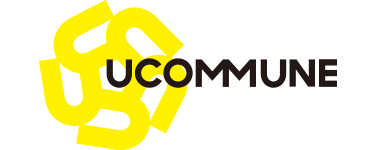
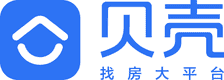


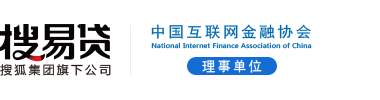
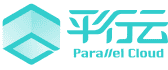


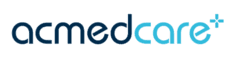
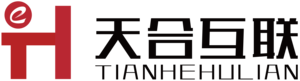


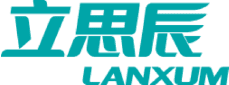
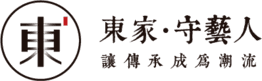





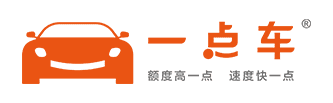

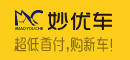
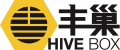
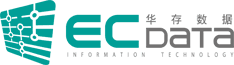
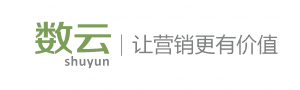

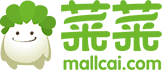
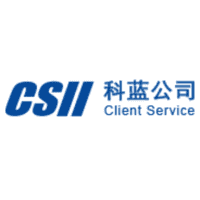
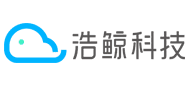

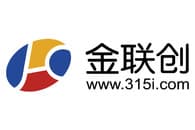
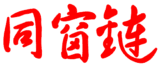
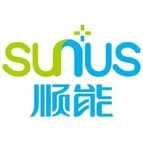
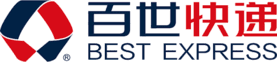
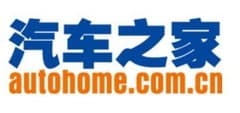
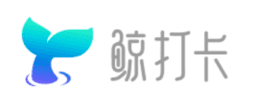
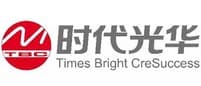
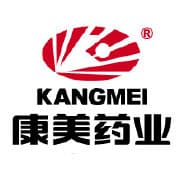
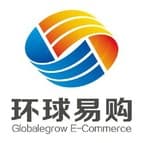

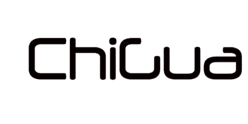
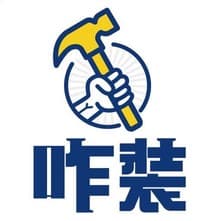

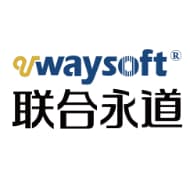
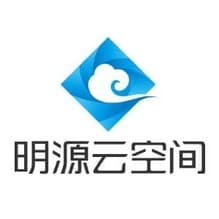

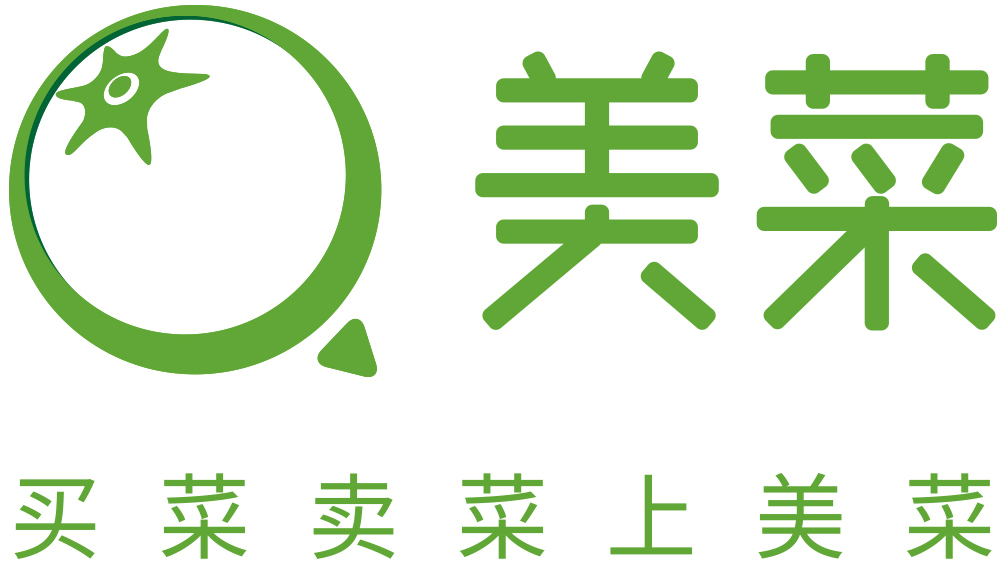
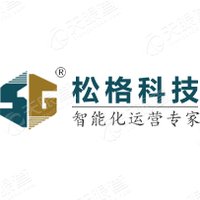



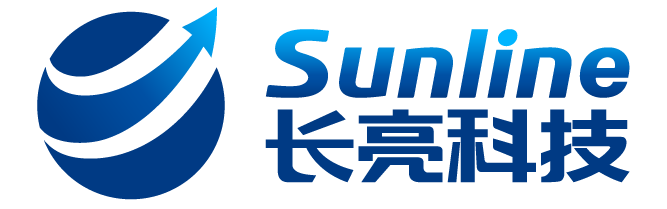





郑州山水, 知氏教育
| 0 |
micrometer-metrics/micrometer | An application observability facade for the most popular observability tools. Think SLF4J, but for observability. | cloud-native java metrics microservices monitoring observability | # Micrometer Application Metrics
[](https://circleci.com/gh/micrometer-metrics/micrometer)
[](https://www.apache.org/licenses/LICENSE-2.0)
[](https://search.maven.org/artifact/io.micrometer/micrometer-core)
[](https://javadocs.dev/io.micrometer)
[](https://ge.micrometer.io/)
An application metrics facade for the most popular monitoring tools. Instrument your code with dimensional metrics with a
vendor neutral interface and decide on the monitoring backend at the last minute.
More info on [micrometer.io](https://micrometer.io).
Micrometer artifacts work with Java 8 or later with a few exceptions such as the micrometer-java11 module and micrometer-jetty11.
## Supported versions
See [Micrometer's support policy](https://micrometer.io/support/).
## Join the discussion
Join the [Micrometer Slack](https://slack.micrometer.io) to share your questions, concerns, and feature requests.
## Snapshot builds
Snapshots are published to `repo.spring.io` for every successful build on the `main` branch and maintenance branches.
To use:
```groovy
repositories {
maven { url 'https://repo.spring.io/snapshot' }
}
dependencies {
implementation 'io.micrometer:micrometer-core:latest.integration'
}
```
## Milestone releases
Milestone releases are published to https://repo.spring.io/milestone.
Include that as a maven repository in your build configuration to use milestone releases.
Note that milestone releases are for testing purposes and are not intended for production use.
## Documentation
The reference documentation is managed in the [docs directory](/docs) and published to https://micrometer.io/.
## Contributing
See our [Contributing Guide](CONTRIBUTING.md) for information about contributing to Micrometer.
-------------------------------------
_Licensed under [Apache Software License 2.0](https://www.apache.org/licenses/LICENSE-2.0)_
_Sponsored by [VMware](https://tanzu.vmware.com)_
| 0 |
luxiaoxun/NettyRpc | A simple RPC framework based on Netty, ZooKeeper and Spring | netty rpc-framework spring zookeeper | # NettyRpc
An RPC framework based on Netty, ZooKeeper and Spring
中文详情:[Chinese Details](http://www.cnblogs.com/luxiaoxun/p/5272384.html)
### Features:
* Simple code and framework
* Service registry/discovery support by ZooKeeper
* High availability, load balance and failover
* Support different load balance strategy
* Support asynchronous/synchronous call
* Support different versions of service
* Support different serializer/deserializer
* Dead TCP connection detecting with heartbeat
### Design:

### How to use (netty-rpc-test)
1. Define an interface:
```
public interface HelloService {
String hello(String name);
String hello(Person person);
}
```
2. Implement the interface with annotation @NettyRpcService:
```
@NettyRpcService(HelloService.class, version = "1.0")
public class HelloServiceImpl implements HelloService {
public HelloServiceImpl(){}
@Override
public String hello(String name) {
return "Hello " + name;
}
@Override
public String hello(Person person) {
return "Hello " + person.getFirstName() + " " + person.getLastName();
}
}
```
3. Run zookeeper
For example: zookeeper is running on 127.0.0.1:2181
4. Start server:
1. Start server with spring config: RpcServerBootstrap
2. Start server without spring config: RpcServerBootstrap2
5. Call the service:
1. Use the client:
```
final RpcClient rpcClient = new RpcClient("127.0.0.1:2181");
// Sync call
HelloService helloService = rpcClient.createService(HelloService.class, "1.0");
String result = helloService.hello("World");
// Async call
RpcService client = rpcClient.createAsyncService(HelloService.class, "2.0");
RPCFuture helloFuture = client.call("hello", "World");
String result = (String) helloFuture.get(3000, TimeUnit.MILLISECONDS);
```
2. Use annotation @RpcAutowired:
```
public class Baz implements Foo {
@RpcAutowired(version = "1.0")
private HelloService helloService1;
@RpcAutowired(version = "2.0")
private HelloService helloService2;
@Override
public String say(String s) {
return helloService1.hello(s);
}
}
``` | 0 |
jhy/jsoup | jsoup: the Java HTML parser, built for HTML editing, cleaning, scraping, and XSS safety. | css css-selectors dom html java java-html-parser jsoup parser xml xpath | # jsoup: Java HTML Parser
**jsoup** is a Java library that makes it easy to work with real-world HTML and XML. It offers an easy-to-use API for URL fetching, data parsing, extraction, and manipulation using DOM API methods, CSS, and xpath selectors.
**jsoup** implements the [WHATWG HTML5](https://html.spec.whatwg.org/multipage/) specification, and parses HTML to the same DOM as modern browsers.
* scrape and [parse](https://jsoup.org/cookbook/input/parse-document-from-string) HTML from a URL, file, or string
* find and [extract data](https://jsoup.org/cookbook/extracting-data/selector-syntax), using DOM traversal or CSS selectors
* manipulate the [HTML elements](https://jsoup.org/cookbook/modifying-data/set-html), attributes, and text
* [clean](https://jsoup.org/cookbook/cleaning-html/safelist-sanitizer) user-submitted content against a safe-list, to prevent XSS attacks
* output tidy HTML
jsoup is designed to deal with all varieties of HTML found in the wild; from pristine and validating, to invalid tag-soup; jsoup will create a sensible parse tree.
See [**jsoup.org**](https://jsoup.org/) for downloads and the full [API documentation](https://jsoup.org/apidocs/).
[](https://github.com/jhy/jsoup/actions?query=workflow%3ABuild)
## Example
Fetch the [Wikipedia](https://en.wikipedia.org/wiki/Main_Page) homepage, parse it to a [DOM](https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model/Introduction), and select the headlines from the *In the News* section into a list of [Elements](https://jsoup.org/apidocs/org/jsoup/select/Elements.html):
```java
Document doc = Jsoup.connect("https://en.wikipedia.org/").get();
log(doc.title());
Elements newsHeadlines = doc.select("#mp-itn b a");
for (Element headline : newsHeadlines) {
log("%s\n\t%s",
headline.attr("title"), headline.absUrl("href"));
}
```
[Online sample](https://try.jsoup.org/~LGB7rk_atM2roavV0d-czMt3J_g), [full source](https://github.com/jhy/jsoup/blob/master/src/main/java/org/jsoup/examples/Wikipedia.java).
## Open source
jsoup is an open source project distributed under the liberal [MIT license](https://jsoup.org/license). The source code is available on [GitHub](https://github.com/jhy/jsoup).
## Getting started
1. [Download](https://jsoup.org/download) the latest jsoup jar (or add it to your Maven/Gradle build)
2. Read the [cookbook](https://jsoup.org/cookbook/)
3. Enjoy!
### Android support
When used in Android projects, [core library desugaring](https://developer.android.com/studio/write/java8-support#library-desugaring) with the [NIO specification](https://developer.android.com/studio/write/java11-nio-support-table) should be enabled to support Java 8+ features.
## Development and support
If you have any questions on how to use jsoup, or have ideas for future development, please get in touch via [jsoup Discussions](https://github.com/jhy/jsoup/discussions).
If you find any issues, please file a [bug](https://jsoup.org/bugs) after checking for duplicates.
The [colophon](https://jsoup.org/colophon) talks about the history of and tools used to build jsoup.
## Status
jsoup is in general, stable release.
| 0 |
mission-peace/interview | Interview questions | null |
<h2>Please visit my wiki link for full list of questions</h2>
<h3>https://github.com/mission-peace/interview/wiki</h3>
<h2> Like my facebook page for latest updates on my youtube channel</h2>
<h3>https://www.facebook.com/tusharroy25</h3>
<h2> Contribution </h2>
Please contribute to this repository to help it make better. Any change like new question, code improvement, doc improvement etc. is very welcome. Just send me a pull request and I will review the request and approve it if it looks good.
<h2> How to use this repository </h2>
<h3> Softwares to install </h3>
* Install JDK8 https://docs.oracle.com/javase/8/docs/technotes/guides/install/install_overview.html
* Install Git https://git-scm.com/book/en/v2/Getting-Started-Installing-Git
* Install either Intellij https://www.jetbrains.com/idea/download/
* If you like eclipse instead of intellij install eclipse https://eclipse.org/downloads/
<h3> Set up your desktop </h3>
* Pull the git repository. Go to command line and type git clone https://github.com/mission-peace/interview.git
* Go to root directory of checked out project.
* Run ./gradlew idea to generate idea related classes
* Fire up intellij. Go to Open. Go to git repo folder and open interview.ipr . On file menu go to project structure. Update language level support to 8
* If you use eclipse, do ./gradlew eclipse . This will generate eclipse related files. Go to eclipse and open up folder containing this repo.
* Go to any program and run that program
* Go to any test and run the junit test.
* Run ./gradlew build to create classes, run tests and create jar.
| 0 |
fenixsoft/jvm_book | 《深入理解Java虚拟机(第3版)》样例代码&勘误 | book jvm | # 《深入理解Java虚拟机(第3版)》
> **广告**:<br/>
> 《[凤凰架构:构建可靠的大型分布式系统](https://icyfenix.cn)》:[https://github.com/fenixsoft/awesome-fenix](https://github.com/fenixsoft/awesome-fenix)
>
> 我的一本新书,也是一部以“讨论如何构筑一套可靠的分布式大型软件系统”为主题的免费开源文档,如对您有用,望不吝给个Star
>
<br/>
本工程为《深入理解Java虚拟机(第3版)》书中的样例代码,以方便读者自行测试。部分代码需要在特定的虚拟机版本、参数下运行,在代码前均已“VM Args”的注释进行标注。
书中的勘误也会在本文中持续更新,读者可通过issues提交新的勘误,如对新版有任何建议,也欢迎以[issues](https://github.com/fenixsoft/jvm_book/issues)或任何其他您方便的形式提出。
### 勘误列表:
- **Page 120**:图3-23应该是【Parallel】,但是印了【Paraller】,差了一个字母
- **Page 219**:Java代码在进行Javac编译的时候,并不像C和C++那样有“连接”这一步骤,而是在虚拟机加载Class文件的时候进行动态连接(具体见【第7章】)。
<br/>更正:Java代码在进行Javac编译的时候,并不像C和C++那样有“连接”这一步骤,而是在虚拟机加载Class文件的时候进行动态连接(具体见【第8章】)。
- **Page 232**:表6-13,JDK 8中新增的属性,用于支持(编译时加上-parameters参数)将【方法名称】编译进Class文件中,并可运行时获取。此前要获取【方法名称】(典型的如IDE的代码提示)只能通过JavaDoc中得到。
<br/>更正:JDK 8中新增的属性,用于支持(编译时加上-parameters参数)将【方法参数名称】编译进Class文件中,并可运行时获取。此前要获取【方法参数名称】(典型的如IDE的代码提示)只能通过JavaDoc中得到。
#### 以下勘误内容已在第10次重印版(2021-12-14日)修正
------
- **Page 223**:表6-6的描述列中,有三处【CONSTANT_NameAndType】,均应为:【CONSTANT_NameAndType_info】
- **page 279**,那就可能造成多个【进程】阻塞
<br/>更正:那就可能造成多个【线程】阻塞
- **Page 295**:图8-1中,【操作栈】应为【操作数栈】,此格的英文【Oper and Stack】应为【Operand Stack】(Operand是一个完整单词)
- **Page 464**:如【图12-11】所示
<br/>更正:如【图12-7】所示
- **书背面**:[#148](https://github.com/fenixsoft/jvm_book/issues/148) 中有同学反映:有评语是“望其项背”,“望其项背”是追得上赶得上,他这意思就相当于其他书可以追得上和赶得上了。
<br/>请编辑从语文专业角度看看这个issue是否正确。
#### 以下勘误内容已在第9次重印版(2021-9-23日)修正(第8次重影因时间关系未修正任何勘误)
------
- **前言VII** 为了实现JDK11新增的【嵌套内】
<br/>更正:为了实现JDK11新增的【嵌套类】
- **目录XIV,Page164** 4.3.3 VisualVM:多合 - 故障处理工具中”的【多合 - 】应该是【多合一】
<br/>这个在原稿上是多合一,但印刷后确实怎么看都像是“多合 - ”,请编辑看看。
- **Page 34**:其中lib的可选值包括有:【boot-jd】、freetype、cups、x、alsa、libffi、jtreg、libjpeg、giflib、libpng、lcms、zlib
<br>更正:其中lib的可选值包括有:【boot-jdk】、freetype、cups、x、alsa、libffi、jtreg、libjpeg、giflib、libpng、lcms、zlib
- **Page 38**:图1-11 在【NetBeans】中创建HotSpot项目(2)
<br>更正:图1-11 在【CLion】中创建HotSpot项目(2)
- **Page 124**:JDK 9之后使用【-X-log:gc*】
<br>更正:JDK 9之后使用【-Xlog:gc*】
- **Page 202**:如果使用【客户端】模式的虚拟机启动Eclipse将会使用到C2编译器
<br>更正:如果使用【服务端】模式的虚拟机启动Eclipse将会使用到C2编译器
- **Page 235**:常量表部分的输出见代码清单【6-1】,因版面原因这里省略掉
<br>更正:常量表部分的输出见代码清单【6-2】,因版面原因这里省略掉
- **Page 246**:常量池在该索引【出】必须是以下结构之一
<br>更正:常量池在该索引【处】必须是以下结构之一
- **Page 259**:代码部分排版有问题:
```
FromTo Target Type
4 10 13 any
13 16 13 any
```
应为:
```
From To Target Type
4 10 13 any
13 16 13 any
```
- **Page 282**:代码清单7-9所示为【java.lang.ClassLoader.getClassLoader()】方法的代码片段
<br>更正:代码清单7-9所示为【java.lang.Class.getClassLoader()】方法的代码片段
- **Page 282**:代码清单7-9 【ClassLoader.getClassLoader()】方法的代码片段
<br>更正:代码清单7-9 【Class.getClassLoader()】方法的代码片段
- **Page 286**:在OSGI环境下,类加载器【不再】双亲委派模型推荐的树状结构
<br>更正:在OSGI环境下,类加载器【不再使用】双亲委派模型推荐的树状结构
- **Page 378**:我们就只有使用【JSR-296】中定义的插入式注解处理器API来对Java编译子系统的行为施加影响
<br>更正:我们就只有使用【JSR-269】中定义的插入式注解处理器API来对Java编译子系统的行为施加影响
#### 以下勘误内容已在第7次重印版(2021-1-11日)修正
------
- **#7-1 Page 99**:作为CMS收集器的替代者和继承人,设计者们希望做出一款能够建立起“【停顿时间模型】”(Pause Prediction Model)的收集器,【停顿时间模型】的意思是能够支持指定在一个长度为M毫秒的时间片段内
<br>全书术语统一为:作为CMS收集器的替代者和继承人,设计者们希望做出一款能够建立起“【停顿预测模型】”(Pause Prediction Model)的收集器,【停顿预测模型】的意思是能够支持指定在一个长度为M毫秒的时间片段内
- **#7-1 Page 85 & 87**:两处代码部分:<br>
`CARD_TABLE [this address >> 9] = 0;`和`if (CARD_TABLE [this address >> 9] != 0) CARD_TABLE [this address >> 9] = 0;`
<br>由于书中文字是以“1标志变脏,0标志未变脏”来描述的,代码中应该统一起来,因此修改为:
<br>`CARD_TABLE [this address >> 9] = 1;`和`if (CARD_TABLE [this address >> 9] != 1) CARD_TABLE [this address >> 9] = 1;`
- **#7-2 Page 108**:我们再来聊一下Shenandoah用以支持【并行整理】的核心概念
<br>更正:我们再来聊一下Shenandoah用以支持【并发整理】的核心概念
- **#7-3 Page 128**:表3-4,最后一行“ParallelGCThreads”的重复了,请编辑删除掉此行,另外这个表格中有两个参数是以“=n”结尾的,为了格式统一,请将“=n”删除掉,仅保留参数名称即可。
- **#7-4 Page 182**:服务器的硬件为四路【志强】处理器
<br>更正:服务器的硬件为四路【至强】处理器
- **#7-5 Page 230**:脚注:Java代码的方法特征签名只包括了方法名称、【参数顺序及参数类型】
<br>更正:Java代码的方法特征签名只包括了方法名称、【参数数量、参数顺序及参数类型】
- **#7-6 Page 268**:加载阶段既可以使用Java虚拟机里内置的【引导类加载器】来完成
<br>全书术语统一为:加载阶段既可以使用Java虚拟机里内置的【启动类加载器】来完成
- **#7-7 Page 268**:Java虚拟机将会把数组C标记为与【引导类加载器】关联。
<br>全书术语统一为:Java虚拟机将会把数组C标记为与【启动类加载器】关联。
- **#7-8 Page 282**:如果需要把加载请求委派给【引导类加载器】去处理
<br>全书术语统一为:如果需要把加载请求委派给【启动类加载器】去处理
- **#7-9 Page 282**:其中的注释和代码实现都明确地说明了以null值来代表【引导类加载器】的约定规则
<br>全书术语统一为:其中的注释和代码实现都明确地说明了以null值来代表【启动类加载器】的约定规则
- **#7-10 Page 448**:这种操作相当于对缓存中的变量做了一次前面介绍Java内存【模式】中所说的“store和write”操作
<br>更正:这种操作相当于对缓存中的变量做了一次前面介绍Java内存【模型】中所说的“store和write”操作
- **#7-11 Page 479**:如适应性自旋(Adaptive Spinning)、锁削除(Lock Elimination)、【锁膨胀】(Lock Coarsening)、轻量级锁(Lightweight Locking)、偏向锁(Biased Locking)等等
<br>更正:如适应性自旋(Adaptive Spinning)、锁削除(Lock Elimination)、【锁粗化】(Lock Coarsening)、轻量级锁(Lightweight Locking)、偏向锁(Biased Locking)等等
- **#7-12 Page 483**:【同时】使用CAS操作把获取到这个锁的线程的ID记录在对象的Mark Word之中
<br>更正:【并】使用CAS操作把获取到这个锁的线程的ID记录在对象的Mark Word之中
#### 以下勘误内容已在第6次重印版(2020-11-5日)修正
------
- **#6-1 Page 46**:到了JDK 7的HotSpot,已经把原本放在永久代的字符串常量池、静态变量等【移出】
<br>更正:到了JDK 7的HotSpot,已经把原本放在永久代的字符串常量池、静态变量等【移至Java堆中,在4.3.1节会通过实验验证这一点】
- **#6-2 Page 70**:在虚拟机栈(栈帧中的本地变量表)中引用的对象,譬如【各个线程被调用的方法堆栈中使用到的参数、局部变量、临时变量等】
<br>有同学反应太过拗口,修改为:在虚拟机栈(栈帧中的本地变量表)中的引用的对象,譬如【当前正在运行的方法所使用到的参数、局部变量、临时变量等】
- **#6-3 Page 94**:-XX:GCTimeRatio参数的值则应当是一个大于0小于100的整数,也就是垃圾收集时间占总时间的比率,这个参数设定为N的话,表示用户代码执行时间与总执行时间之比为N:N+1。譬如把此参数设置为19,那允许的最大垃圾收集时间就占总时间的5%(即1 /(1+19)),默认值为99,就是允许最大1%(即1 /(1+99))的垃圾收集时间。
<br>整一段修正为:-XX:GCTimeRatio参数的值应为设置为一个正整数,表示用户期望虚拟机消耗在GC上的时间不超过程序运行时间的1/(1+N)。默认值为99,含义是尽可能保证应用程序执行的时间为收集器执行时间的99倍,也即是收集器的时间消耗不超过总运行时间的1%。
- **#6-4 Page 243**:表6-26,第6行:ACC_INTERFACE 【0x0020】
<br>更正:ACC_INTERFACE 【0x0200】
- **#6-5 Page 256**:如果v在目标类型T(int或long)的表示范围之【类】
<br>更正:如果v在目标类型T(int或long)的表示范围之【内】
- **#6-6 Page 274**:接着由虚拟机生成一个代表该数组维度和元素的【数组对象】。
<br>更正:接着由虚拟机生成一个代表该数组维度和元素的【数组类型】。
- **#6-7 Page 317**:本例中为一项【CONSTANT_InterfaceMethodref_info】常量
<br>更正:本例中为一项【CONSTANT_Methodref_info】常量
- **#6-8 Page 331**:图8-6 执行偏移地址为【1】的指令的情况
<br>更正:图8-6 执行偏移地址为【2】的指令的情况
- **#6-9 Page 385**:代码清单10-18 第13行需修改方法名称,以符合输出结果:
<br>`protected void BADLY_NAMED_CODE()` { 修改为 `protected void Test() {`
- **#6-10 Page 392**:脚注:还有一个不太上台面但其实是Java虚拟机必须支持循环体触发编译的【理由,是】诸多跑分软件的测试用例通常都属于第二种,如果不去支持跑分会显得成绩很不好看。
<br>更正为:还有一个不太上台面但其实是Java虚拟机必须支持循环体触发编译的【理由是:】诸多跑分软件的测试用例通常都属于第二种,如果不去支持跑分会显得成绩很不好看。
- **#6-11 Page 419**:p.y = 42
<br>更正:p.y = 42【;】
- **#6-12 Page 420**:int py = 42
<br>更正:int py = 42【;】
- **#6-13 Page 431**:与【图11-11】和【图11-12】所示相比,虽然没有了箭头
<br>更正:与【图11-13】和【图11-14】所示相比,虽然没有了箭头
- **#6-14 Page 443**:Java内存模型的基础设计并未改变,即使是【这四操作种】对普通用户阅读使用起来仍然是并不方便。
<br>更正:Java内存模型的基础设计并未改变,即使是【这四种操作】对普通用户阅读使用起来仍然是并不方便。
- **#6-15 Page 467**:在【第10章】里我们讲解“final关键字带来的可见性”时曾经提到过这一点
<br>更正:在【第12章】里我们讲解“final关键字带来的可见性”时曾经提到过这一点
- **#6-16 Page 472**:在【第10章】中我们知道了主流Java虚拟机实现中,Java的线程是映射到操作系统的原生内核线程之上的
<br>更正:在【第12章】中我们知道了主流Java虚拟机实现中,Java的线程是映射到操作系统的原生内核线程之上的
- **#6-17 Page 480**:锁削除是指虚拟机即时编译器在运行时,对一些代码中要求同步,但是被检测到不可能存在共享数据竞争的锁进行削除。
<br>这句话有读者反应不通顺,整句修改为:锁消除是指虚拟机即时编译器在运行时检测到某段需要同步的代码根本不可能存在共享数据竞争而实施的一种对锁进行消除的优化策略。
#### 以下勘误内容已在第5次重印版(2020-9-11日)修正
------
- **#5-1 Page 51**:以下代码清单2-2为HotSpot虚拟机代表Mark Word中的代码(【markOop.cpp】)注释片段
<br>更正:以下代码清单2-2为HotSpot虚拟机代表Mark Word中的代码(【markOop.hpp】)注释片段。另,下面代码清单2-2也应更正为“代码清单2-2 【markOop.hpp】片段”
- **#5-2 Page 72**:要真正宣告一个对象死亡,【至少要】经历两次标记过程
<br>更正:要真正宣告一个对象死亡,【最多会】经历两次标记过程
- **#5-3 Page 78**:图3-2、图3-3,原稿中第2行2列、3行2列、3行4列都是“可回收”对象的颜色,排版重画的时候颜色搞错了,麻烦编辑下次印刷中修改过来。
- **#5-4 Page 79**:发生垃圾【搜集】时,将Eden和Survivor中仍然存活的对象一次性过拷贝到另外一块Survivor空间上
<br>更正:发生垃圾【收集】时,将Eden和Survivor中仍然存活的对象一次性过拷贝到另外一块Survivor空间上
- **#5-5 Page 94**:-XX:GCTimeRatio参数的值则应当是一个大于0小于100的整数,也就是垃圾收集时间占总时间的比率,【相当于是吞吐量的倒数】。
<br>更正:-XX:GCTimeRatio参数的值则应当是一个大于0小于100的整数,也就是垃圾收集时间占总时间的比率,【这个参数设定为N的话,表示用户代码执行时间与总执行时间之比为N:N+1】
- **#5-6 Page 95**:Parallel Old是Parallel Scavenge收集器的老年代版本,支持多线程【并发收集】
<br>更正:Parallel Old是Parallel Scavenge收集器的老年代版本,支持多线程【并行收集】
- **#5-7 Page 109**:一旦用户程序访问到归属于旧对象的内存空间就会产生自陷【中段】
<br>更正:一旦用户程序访问到归属于旧对象的内存空间就会产生自陷【中断】
- **#5-8 Page 128**:JDK 9之前虚拟机运行在Server模式下的默认值,打开此开关后,使用【Parallel Scavenge + Serial Old(PS MarkSweep)的】收集器组合进行内存回收
<br>更正:JDK 9之前虚拟机运行在Server模式下的默认值,打开此开关后,使用【Parallel】收集器组合进行内存回收
- **#5-9 Page 215**:Class文件是一组以【8个字节】为基础单位的二进制流,各个数据项目严格按照顺序紧凑地排列在文件之中,中间没有添加任何分隔符,这使得整个Class文件中存储的内容几乎全部是程序运行的必要数据,没有空隙存在。当遇到需要占用【8个字节】以上空间的数据项时,则会按照高位在前 的方式分割成若干个【8个字节】进行存储。
<br>更正:Class文件是一组以【字节】为基础单位的二进制流,各个数据项目严格按照顺序紧凑地排列在文件之中,中间没有添加任何分隔符,这使得整个Class文件中存储的内容几乎全部是程序运行的必要数据,没有空隙存在。当遇到需要占用【单个字节】以上空间的数据项时,则会按照高位在前 的方式分割成若干个【字节】进行存储。
- **#5-10 Page 259**:3 monitorenter // 以【栈定】元素(即f)作为锁,开始同步
<br>更正:3 monitorenter // 以【栈顶】元素(即f)作为锁,开始同步
- **#5-11 Page 265**:上述代码运行之后,只会输出“SuperClass init!”
<br>更正:上述代码运行之后,【除value的值外,】只会输出“SuperClass init!”
- **#5-12 Page 280**:成为了Java技术体系中一块重要的基石,可谓是【失之桑榆,收之东隅】。
<br>更正:成为了Java技术体系中一块重要的基石,可谓是【失之东隅,收之桑榆】。
- **#5-13 Page 307**:'a'除了可以代表一个【字符串】
<br>更正:'a'除了可以代表一个【字符】
- **#5-14 Page 392**:脚注:是诸多跑分软件的测试【用力】通常都属于第二种,如果不去支持跑分会显得成绩很不好看。
<br>更正:是诸多跑分软件的测试【用例】通常都属于第二种,如果不去支持跑分会显得成绩很不好看。
- **#5-15 Page 432**:在Outline视图中找到创建理想图的方法是【greateGraph()】
<br>更正:在Outline视图中找到创建理想图的方法是【createGraph()】(这个笔误在432页一共有3处)
- **#5-16 Page 472**:脚注2:由于本书的主题是Java虚拟机【和】不是Java并发编程
<br>更正:由于本书的主题是Java虚拟机【而】不是Java并发编程
- **#5-17 Page 480**:对一些代码要求同步,但是【对被检测】到不可能存在共享数据竞争的锁进行消除
<br>更正:对一些代码要求同步,但是【虚拟机又检测】到不可能存在共享数据竞争的锁进行消除
#### 以下勘误内容已在第4次重印版(2020-6-10日)修正
------
- **#4-1 Page 25**:即不能动态加载其他【编译器】不可知的代码和类库
<br>更正:即不能动态加载其他【编译期】不可知的代码和类库
- **#4-2 Page 30**:JDK 7则【还完全】处于研发状态的半成品。
<br>更正:JDK 7则【完全是】处于研发状态的半成品。
- **#4-3 Page 38**:由于JDK12已EOL,RedHat的GitHub上目前只维护8、11两个LTS和13这个正在半年支持期内的JDK版本,JDK12的Repo已经关闭(但从Git的History中挖掘出来是很容易的),为了便于读者获取 CMakeLists.txt,我上传了一份到本仓库中。此文件版权归原作者所有。(编辑在更新勘误时可跳过此条)
- **#4-4 Page 48**:Java是一门面向对象的编程语言,Java程序运行过程中【无时无刻都】有对象被创建出来
<br>更正:Java是一门面向对象的编程语言,Java程序运行过程中【每时每刻都】有对象被创建出来
- **#4-5 Page 58-60**:代码清单2-4、2-5的输出,在代码中定义的方法名是“stackLeak()”,在输出日志中看到的堆栈的方法名是“leak()”。这个是代码粘贴后整理编辑过方法名,不影响意思表达。(方法名在日志、代码中出现次数较多,编辑处理这条勘误时请单独联系我提供更正详情)
- **#4-6 Page 80**:HotSpot虚拟机里面关注吞吐量的Parallel 【Scavenge】收集器是基于标记-整理算法的。
<br>更正:HotSpot虚拟机里面关注吞吐量的Parallel 【Old】收集器是基于标记-整理算法的。
- **#4-7 Page 97**:并发回收时垃圾收集线程【只占用不超过25%】的处理器运算资源,并且会随着处理器核心数量的增加而下降。
<br>更正:并发回收时垃圾收集线程【占用不少于25%】的处理器运算资源,并且会随着处理器核心数量的增加而下降。
- **#4-8 Page 105**:图3-14中第3行,G1收集器的Compact阶段应该为【浅色】,这个我交上去的原图是正确的,但编辑重新画图时候涂层了深色。请编辑更新新版的时候注意一下。
- **#4-9 Page 106**:在回收时通过这张表格就可以得出哪些Region之间产生了【跨代引用】。
<br>更正:在回收时通过这张表格就可以得出哪些Region之间产生了【跨Region的引用】。
- **#4-10 Page 107**:图3-15中,两个X的位置打错了,应该在5行3列、3行1列处打X
- **#4-11 Page 106**:降低了处理跨代指针时的记忆集维护消耗,也降低了伪共享问题(【见3.4.4节】)的发生概率。
<br>更正:降低了处理跨代指针时的记忆集维护消耗,也降低了伪共享问题(【见3.4.5节】)的发生概率。
- **#4-12 Page 134**:如果在Survivor空间中【相同年龄】所有对象大小的总和大于Survivor空间的一半
<br>更正:如果在Survivor空间中【低或等于某个年龄的】所有对象大小的总和大于Survivor空间的一半
- **#4-13 Page 134**:满足【同年】对象达到Survivor空间的一半规则
<br>更正:满足【低于或等于某年龄的】对象达到Survivor空间的一半规则
- **#4-14 Page 162-163**:代码中类名“SynAddRunalbe”中英文“Runalbe”为拼写错误,应为“SynAddRunnable”,代码中一共有4处,图片中有1处。
- **#4.15 Page 239**:Exceptions属性的作用是列举出方法中可能抛出的受查异常(Checked 【Excepitons】)
<br>更正:Exceptions属性的作用是列举出方法中可能抛出的受查异常(Checked 【Exceptions】)
- **#4.16 Page 265**:创建动作由字节码指令【newarray】触发
<br>更正:创建动作由字节码指令【anewarray】触发
- **#4-17 Page 272**:【JDK 7及之前】,HotSpot使用永久代来实现方法区时,实现是完全符合这种逻辑概念的;【而在JDK 8及之后】,类变量则会随着Class对象一起存放在Java堆中
<br>更正:【JDK 7之前】,HotSpot使用永久代来实现方法区时,实现是完全符合这种逻辑概念的;【而在JDK 7及之后】,类变量则会随着Class对象一起存放在Java堆中
- **#4-18 Page 274**:被访问类C是public的,不与访问类D处于同一个模块,但是被访问类C的模块允许【被访问类D】的模块进行访问。
<br>更正:被访问类C是public的,不与访问类D处于同一个模块,但是被访问类C的模块允许【访问类D】的模块进行访问。
- **#4-19 Page 312**:运行结果的第3行:This 【gay】 has $2
<br>更正:This 【guy】 has $2
- **#4-20 Page 314**:脚注三最后一行:具体可【常见】第11章关于方法内联的内容。
<br>更正:具体可【参见】第11章关于方法内联的内容。
- **#4-21 Page 461**:图12-6,New->Running,Running->Terminated这两个是单向箭头,交给编辑的原稿上也还是单向的,应该是排版修图时搞错变成双向了,请编辑同学修正过来。
- **#4-22 Page 462**:【以前处理一个请求可以允许花费很长时间在单体应用中】,具有这种线程切换成本也是无伤大雅的
<br>语句不通,更正为:【在以前的单体应用中,处理一个请求可以允许花费很长时间】,具有这种线程切换成本也是无伤大雅的
- **#4-23 Page 462**:现实的需求在迫使Java去研究新的解决方案,【同】大家又怀念起以前绿色线程的种种好处
<br>更正为:现实的需求在迫使Java去研究新的解决方案,【此时】大家又怀念起以前绿色线程的种种好处
#### 以下勘误内容已在第3次重印版(2020-2-20日)修正
------
- **#3-1 Page 27**:到了JDK 10,HotSpot又重构了Java虚拟机的垃圾收集器接口 (Java Virtual Machine 【Compiler】 Interface)
<br>更正:到了JDK 10,HotSpot又重构了Java虚拟机的垃圾收集器接口 (Java Virtual Machine 【Garbage】 Interface)
- **#3-2 Page 37**:譬如【Eclipst】 CDT或者NetBeans来进行的话
<br>更正:譬如【Eclipse】 CDT或者NetBeans来进行的话
- **#3-3 Page 110**:对象的读取、写入、对象的比较、【为对象哈希值计算】、用对象加锁等等
<br>更正:对象的读取、写入、对象的比较、【为对象计算哈希值】、用对象加锁等等
- **#3-4 Page 113**:图3-19中,【Larage】为笔误,应为【Large】
- **#3-5 Page 238**:13: iload_1 后的注释应该是字节码第14行的,即
> 13: iload_1 // 保存x到returnValue中,此时x=2
> 14: istore 4
改为:
> 13: iload_1
> 14: istore 4 // 保存x到returnValue中,此时x=2
- **#3-6 Page 254**:算术指令用于对【两个操作数栈上的值】进行某种特定运算
<br>对语序修改以避免歧义:算术指令用于对【操作数栈上的两个值】进行某种特定运算
- **#3-7 Page 259**:13 astore_3 后注释【Taget】为笔误,应为【Target】
- **#3-8 Page 265/266**:在266页正文中出现两次注释一,其中第一个注释是265页才对,应该是排版问题,请编辑再版时注意。
- **#3-9 Page 278**:代码清单7-5,第一行注释:给变量【复制】可以正常编译通过
<br>更正:
给变量【赋值】可以正常编译通过
- **#3-10 Page 278**:代码清单7-5,第二行注释:这句编译器会提示“非法【向前】引用”
<br>更正:
这句编译器会提示“非法【前向】引用”
- **#3-11 Page 290**:用在IntelliJ 【IDE】、Eclipse这些IDE上做HotSwap……
<br>更正:
用在IntelliJ 【IDEA】、Eclipse这些IDE上做HotSwap……
- **#3-12 Page 312**:代码实例中出现三处【gay】,譬如Father gay = new Son(); 均应为【guy】,这个不影响代码运行,只是不太雅观。
- **#3-13 Page 317**:产生这种差别产生的根本原因是Java语言在编译期间【却】已将println(String)方法完整的符号引用。
<br>更正:产生这种差别产生的根本原因是Java语言在编译期间【就】已将println(String)方法完整的符号引用。
- **#3-14 Page 322**:由于注解中John Rose博客文章中的代码托管网站Kenai.com已经关闭,为了便于读者获取INDY工具,我上传了一份到本仓库中(源码,在src/indify目录)。此文件版权归原作者John Rose所有。(编辑在更新勘误时可跳过此条)
- **#3-15 Page 348**:通常解决【类】问题有以下几种途径
<br>更正:通常解决【此类】问题有以下几种途径
- **#3-16 Page 372**:譬如将【代码清单10-2】稍微修改一下,变成下面代码清单10-7这个样子
<br>更正:譬如将【代码清单10-4】稍微修改一下,变成下面代码清单10-7这个样子
- **#3-17 Page 396**:【图11-2】和【图11-3】都仅仅是描述了客户端模式虚拟机的即时编译方式
<br>更正:【图11-3】和【图11-4】都仅仅是描述了客户端模式虚拟机的即时编译方式
#### 以下勘误内容已在第2次重印版(2019-12-27日)修正
------
- **#2.1 前言部分**:读者反馈信箱:understandjvm@gmail.com
<br>更正:由于这个信箱由于一直只收未发,刚印刷后收到Google的通知此账号已自动作废。而且根据Google规定,作废后无法注册同名邮箱。下次重印将修改为本工程地址:https://github.com/fenixsoft/jvm_book。
- **#2-2 Page 9**:支持HTTP 2客户【单】API等91个JEP
<br>更正:支持HTTP 2客户【端】API等91个JEP
- **#2-3 Page 64**:在【代码清单2-8】里笔者借助了CGLib……
<br>更正:在【代码清单2-9】里笔者借助了CGLib……
- **#2.4 Page 368**: 【ArrayList<int>】与ArrayList<String>其实是同一个类型
<br>更正:【ArrayList<Integer>】与ArrayList<String>其实是同一个类型
| 0 |
Jzvd/JZVideo | 高度自定义的安卓视频框架 MediaPlayer exoplayer ijkplayer ffmpeg | jiaozi jzt jzvd videoplayer | 高度自定义的安卓视频框架
[中文文档](http://jzvd.org)
[下载Demo](https://github.com/Jzvd/JZVideo/releases/download/v7.7.0/jiaozivideoplayer-7.7.0.apk)
## QuickStart
1.添加类库
```gradle
implementation 'cn.jzvd:jiaozivideoplayer:7.7.0'
```
2.添加布局
```xml
<cn.jzvd.JzvdStd
android:id="@+id/jz_video"
android:layout_width="match_parent"
android:layout_height="200dp" />
```
3.设置视频地址、缩略图地址、标题
```java
MyJzvdStd jzvdStd = (MyJzvdStd) findViewById(R.id.jz_video);
jzvdStd.setUp("http://jzvd.nathen.cn/c6e3dc12a1154626b3476d9bf3bd7266/6b56c5f0dc31428083757a45764763b0-5287d2089db37e62345123a1be272f8b.mp4"
, "饺子闭眼睛");
jzvdStd.posterImageView.setImage("http://p.qpic.cn/videoyun/0/2449_43b6f696980311e59ed467f22794e792_1/640");
```
4.在`Activity`中
```java
@Override
public void onBackPressed() {
if (Jzvd.backPress()) {
return;
}
super.onBackPressed();
}
@Override
protected void onPause() {
super.onPause();
Jzvd.releaseAllVideos();
}
```
5.在`AndroidManifest.xml`中
```
<activity
android:name=".MainActivity"
android:configChanges="orientation|screenSize|keyboardHidden"
android:screenOrientation="portrait" /> <!-- or android:screenOrientation="landscape"-->
```
6.在`proguard-rules.pro`中按需添加
```
-keep public class cn.jzvd.JZMediaSystem {*; }
-keep public class cn.jzvd.demo.CustomMedia.CustomMedia {*; }
-keep public class cn.jzvd.demo.CustomMedia.JZMediaIjk {*; }
-keep public class cn.jzvd.demo.CustomMedia.JZMediaSystemAssertFolder {*; }
-keep class tv.danmaku.ijk.media.player.** {*; }
-dontwarn tv.danmaku.ijk.media.player.*
-keep interface tv.danmaku.ijk.media.player.** { *; }
```
./gradlew build -x androidJavadocs
## 效果
<p align="center">
<img src="https://user-images.githubusercontent.com/2038071/94292970-3fdc5800-ff90-11ea-97df-7b9b7455fe7d.jpg" width="75%">
</p>
<p align="center">
<img src="https://user-images.githubusercontent.com/2038071/76214561-f6245e00-6247-11ea-85bb-da35ede45463.gif" width="75%">
</p>
## License MIT
Copyright (c) 2015-2021 jzvd.org
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
| 0 |
justauth/JustAuth | 🏆Gitee 最有价值开源项目 🚀:100: 小而全而美的第三方登录开源组件。目前已支持Github、Gitee、微博、钉钉、百度、Coding、腾讯云开发者平台、OSChina、支付宝、QQ、微信、淘宝、Google、Facebook、抖音、领英、小米、微软、今日头条、Teambition、StackOverflow、Pinterest、人人、华为、企业微信、酷家乐、Gitlab、美团、饿了么、推特、飞书、京东、阿里云、喜马拉雅、Amazon、Slack和 Line 等第三方平台的授权登录。 Login, so easy! | googlelogin justauth oauth2 qqlogin weixinlogin | <p align="center">
<a href="https://www.justauth.cn"><img src="https://gitee.com/yadong.zhang/static/raw/master/JustAuth/Justauth.png" width="400"></a>
</p>
<p align="center">
<strong>Login, so easy.</strong>
</p>
<p align="center">
<a target="_blank" href="https://search.maven.org/search?q=JustAuth">
<img src="https://img.shields.io/github/v/release/justauth/JustAuth?style=flat-square" ></img>
</a>
<a target="_blank" href="https://oss.sonatype.org/content/repositories/snapshots/me/zhyd/oauth/JustAuth/">
<img src="https://img.shields.io/nexus/s/https/oss.sonatype.org/me.zhyd.oauth/JustAuth.svg?style=flat-square" ></img>
</a>
<a target="_blank" href="https://gitee.com/yadong.zhang/JustAuth/blob/master/LICENSE">
<img src="https://img.shields.io/apm/l/vim-mode.svg?color=yellow" ></img>
</a>
<a target="_blank" href="https://www.oracle.com/technetwork/java/javase/downloads/index.html">
<img src="https://img.shields.io/badge/JDK-1.8+-green.svg" ></img>
</a>
<a target="_blank" href="https://www.justauth.cn" title="参考文档">
<img src="https://img.shields.io/badge/Docs-latest-blueviolet.svg" ></img>
</a>
<a href="https://codecov.io/gh/justauth/JustAuth">
<img src="https://codecov.io/gh/justauth/JustAuth/branch/master/graph/badge.svg?token=zYiAqd9aFz" />
</a>
<a href='https://gitee.com/yadong.zhang/JustAuth/stargazers'>
<img src='https://gitee.com/yadong.zhang/JustAuth/badge/star.svg?theme=gvp' alt='star'></img>
</a>
<a target="_blank" href='https://github.com/zhangyd-c/JustAuth'>
<img src="https://img.shields.io/github/stars/zhangyd-c/JustAuth.svg?style=social" alt="github star"></img>
</a>
</p>
-------------------------------------------------------------------------------
<p align="center">
<img src='./docs/media/75a3c076.png' alt='star'></img>
</p>
-------------------------------------------------------------------------------
QQ 群:230017570
微信群:justauth (备注`justauth`或者`ja`)
帮助文档:[www.justauth.cn](https://www.justauth.cn)
## 什么是 JustAuth?
JustAuth,如你所见,它仅仅是一个**第三方授权登录**的**工具类库**,它可以让我们脱离繁琐的第三方登录 SDK,让登录变得**So easy!**
JustAuth 集成了诸如:Github、Gitee、支付宝、新浪微博、微信、Google、Facebook、Twitter、StackOverflow等国内外数十家第三方平台。更多请参考<a href="https://www.justauth.cn" target="_blank">已集成的平台</a>
## 有哪些特点?
1. **全**:已集成十多家第三方平台(国内外常用的基本都已包含),仍然还在持续扩展中([开发计划](https://gitee.com/yadong.zhang/JustAuth/issues/IUGRK))!
2. **简**:API就是奔着最简单去设计的(见后面`快速开始`),尽量让您用起来没有障碍感!
## 有哪些功能?
- 集成国内外数十家第三方平台,实现快速接入。<a href="https://www.justauth.cn/quickstart/how-to-use.html" target="_blank">参考文档</a>
- 自定义 State 缓存,支持各种分布式缓存组件。<a href="https://www.justauth.cn/features/customize-the-state-cache.html" target="_blank">参考文档</a>
- 自定义 OAuth 平台,更容易适配自有的 OAuth 服务。<a href="https://www.justauth.cn/features/customize-the-oauth.html" target="_blank">参考文档</a>
- 自定义 Http 实现,选择权完全交给开发者,不会单独依赖某一具体实现。<a href="https://www.justauth.cn/quickstart/how-to-use.html#%E4%BD%BF%E7%94%A8%E6%96%B9%E5%BC%8F" target="_blank">参考文档</a>
- 自定义 Scope,支持更完善的授权体系。<a href="https://www.justauth.cn/features/customize-scopes.html" target="_blank">参考文档</a>
- 更多...<a href="https://www.justauth.cn" target="_blank">参考文档</a>
## 快速开始
### 引入依赖
```xml
<dependency>
<groupId>me.zhyd.oauth</groupId>
<artifactId>JustAuth</artifactId>
<version>{latest-version}</version>
</dependency>
```
> **latest-version** 可选:
> - 稳定版:
> - 快照版:
> > 注意:快照版本是功能的尝鲜,并不保证稳定性。请勿在生产环境中使用。
>
> <details>
> <summary>如何引入快照版本</summary>
>
> JustAuth 的快照版本托管在 ossrh 上,所以要指定下载地址。
>
> ```xml
> <repositories>
> <repository>
> <id>ossrh-snapshot</id>
> <url>https://oss.sonatype.org/content/repositories/snapshots</url>
> <snapshots>
> <enabled>true</enabled>
> </snapshots>
> </repository>
> </repositories>
> ```
>
> 如果你想第一时间获取 JustAuth 的最新快照,可以添加下列代码,每次构建时都检查是否有最新的快照(默认每天检查)。
>
> ```diff
> <url>https://oss.sonatype.org/content/repositories/snapshots</url>
> <snapshots>
> + <updatePolicy>always</updatePolicy>
> <enabled>true</enabled>
> </snapshots>
> ```
>
> </details>
如下**任选一种** HTTP 工具 依赖,_项目内如果已有,请忽略。另外需要特别注意,如果项目中已经引入了低版本的依赖,请先排除低版本依赖后,再引入高版本或者最新版本的依赖_
- hutool-http
```xml
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-http</artifactId>
<version>5.7.7</version>
</dependency>
```
- httpclient
```xml
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
```
- okhttp
```xml
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>4.9.1</version>
</dependency>
```
### 调用api
#### 普通方式
```java
// 创建授权request
AuthRequest authRequest = new AuthGiteeRequest(AuthConfig.builder()
.clientId("clientId")
.clientSecret("clientSecret")
.redirectUri("redirectUri")
.build());
// 生成授权页面
authRequest.authorize("state");
// 授权登录后会返回code(auth_code(仅限支付宝))、state,1.8.0版本后,可以用AuthCallback类作为回调接口的参数
// 注:JustAuth默认保存state的时效为3分钟,3分钟内未使用则会自动清除过期的state
authRequest.login(callback);
```
#### Builder 方式一
静态配置 `AuthConfig`
```java
AuthRequest authRequest = AuthRequestBuilder.builder()
.source("github")
.authConfig(AuthConfig.builder()
.clientId("clientId")
.clientSecret("clientSecret")
.redirectUri("redirectUri")
.build())
.build();
// 生成授权页面
authRequest.authorize("state");
// 授权登录后会返回code(auth_code(仅限支付宝))、state,1.8.0版本后,可以用AuthCallback类作为回调接口的参数
// 注:JustAuth默认保存state的时效为3分钟,3分钟内未使用则会自动清除过期的state
authRequest.login(callback);
```
#### Builder 方式二
动态获取并配置 `AuthConfig`
```java
AuthRequest authRequest = AuthRequestBuilder.builder()
.source("gitee")
.authConfig((source) -> {
// 通过 source 动态获取 AuthConfig
// 此处可以灵活的从 sql 中取配置也可以从配置文件中取配置
return AuthConfig.builder()
.clientId("clientId")
.clientSecret("clientSecret")
.redirectUri("redirectUri")
.build();
})
.build();
Assert.assertTrue(authRequest instanceof AuthGiteeRequest);
System.out.println(authRequest.authorize(AuthStateUtils.createState()));
```
#### Builder 方式支持自定义的平台
```java
AuthRequest authRequest = AuthRequestBuilder.builder()
// 关键点:将自定义实现的 AuthSource 配置上
.extendSource(AuthExtendSource.values())
// source 对应 AuthExtendSource 中的枚举 name
.source("other")
// ... 其他内容不变,参考上面的示例
.build();
```
## 赞助和支持
感谢以下赞助商的支持:
[我要赞助](https://www.justauth.cn/sponsor.html)
## JustAuth 的用户
有很多公司、组织和个人把 JustAuth 用于学习、研究、生产环境和商业产品中,包括(但不限于):
[](https://www.mochiwang.com "给作者提供云写作的一个工具")

怎么没有我?[登记](https://gitee.com/yadong.zhang/JustAuth/issues/IZ2T7)
## 开源推荐
- `JAP` 开源的登录认证中间件: [https://gitee.com/fujieid/jap](https://gitee.com/fujieid/jap)
- `spring-boot-demo` 深度学习并实战 spring boot 的项目: [https://github.com/xkcoding/spring-boot-demo](https://github.com/xkcoding/spring-boot-demo)
- `mica` SpringBoot 微服务高效开发工具集: [https://github.com/lets-mica/mica](https://github.com/lets-mica/mica)
- `sureness` 面向restful api的高性能认证鉴权框架:[sureness](https://github.com/usthe/sureness)
更多推荐,请参考:[JustAuth - 开源推荐](https://www.justauth.cn)
## 鸣谢
- 感谢 JetBrains 提供的免费开源 License:
<p>
<img src="https://images.gitee.com/uploads/images/2020/0406/220236_f5275c90_5531506.png" alt="图片引用自lets-mica" style="float:left;">
</p>
## 其他
- [CONTRIBUTORS](https://www.justauth.cn/contributors.html)
- [CHANGELOGS](https://www.justauth.cn/update.html)
- [PLAN](https://gitee.com/yadong.zhang/JustAuth/issues/IUGRK)
## 贡献者列表
[](https://whnb.wang)
## Stars 趋势
### Gitee
[](https://whnb.wang/yadong.zhang/JustAuth?e=604800)
### Github
[](https://starchart.cc/justauth/JustAuth)
### ProductHunt
<a href="https://www.producthunt.com/posts/justauth?utm_source=badge-featured&utm_medium=badge&utm_souce=badge-justauth" target="_blank"><img src="https://api.producthunt.com/widgets/embed-image/v1/featured.svg?post_id=196886&theme=dark" alt="JustAuth - Login, so easy! | Product Hunt Embed" style="width: 250px; height: 54px;" width="250px" height="54px" /></a>
| 0 |
amaembo/streamex | Enhancing Java Stream API | collections java java8 streams-api | # StreamEx 0.8.2
Enhancing Java Stream API.
[](https://maven-badges.herokuapp.com/maven-central/one.util/streamex/)
[](https://www.javadoc.io/doc/one.util/streamex)
[](https://github.com/amaembo/streamex/actions/workflows/test.yml)
[](https://coveralls.io/github/amaembo/streamex?branch=master)
This library defines four classes: `StreamEx`, `IntStreamEx`, `LongStreamEx`, `DoubleStreamEx`
which are fully compatible with Java 8 stream classes and provide many additional useful methods.
Also `EntryStream` class is provided which represents the stream of map entries and provides
additional functionality for this case. Finally, there are some new useful collectors defined in `MoreCollectors`
class as well as primitive collectors concept.
Full API documentation is available [here](http://amaembo.github.io/streamex/javadoc/).
Take a look at the [Cheatsheet](wiki/CHEATSHEET.md) for brief introduction to the StreamEx!
Before updating StreamEx check the [migration notes](wiki/MIGRATION.md) and full list of [changes](wiki/CHANGES.md).
StreamEx library main points are following:
* Shorter and convenient ways to do the common tasks.
* Better interoperability with older code.
* 100% compatibility with original JDK streams.
* Friendliness for parallel processing: any new feature takes the advantage on parallel streams as much as possible.
* Performance and minimal overhead. If StreamEx allows to solve the task using less code compared to standard Stream, it
should not be significantly slower than the standard way (and sometimes it's even faster).
### Examples
Collector shortcut methods (toList, toSet, groupingBy, joining, etc.)
```java
List<String> userNames = StreamEx.of(users).map(User::getName).toList();
Map<Role, List<User>> role2users = StreamEx.of(users).groupingBy(User::getRole);
StreamEx.of(1,2,3).joining("; "); // "1; 2; 3"
```
Selecting stream elements of specific type
```java
public List<Element> elementsOf(NodeList nodeList) {
return IntStreamEx.range(nodeList.getLength())
.mapToObj(nodeList::item).select(Element.class).toList();
}
```
Adding elements to stream
```java
public List<String> getDropDownOptions() {
return StreamEx.of(users).map(User::getName).prepend("(none)").toList();
}
public int[] addValue(int[] arr, int value) {
return IntStreamEx.of(arr).append(value).toArray();
}
```
Removing unwanted elements and using the stream as Iterable:
```java
public void copyNonEmptyLines(Reader reader, Writer writer) throws IOException {
for(String line : StreamEx.ofLines(reader).remove(String::isEmpty)) {
writer.write(line);
writer.write(System.lineSeparator());
}
}
```
Selecting map keys by value predicate:
```java
Map<String, Role> nameToRole;
public Set<String> getEnabledRoleNames() {
return StreamEx.ofKeys(nameToRole, Role::isEnabled).toSet();
}
```
Operating on key-value pairs:
```java
public Map<String, List<String>> invert(Map<String, List<String>> map) {
return EntryStream.of(map).flatMapValues(List::stream).invert().grouping();
}
public Map<String, String> stringMap(Map<Object, Object> map) {
return EntryStream.of(map).mapKeys(String::valueOf)
.mapValues(String::valueOf).toMap();
}
Map<String, Group> nameToGroup;
public Map<String, List<User>> getGroupMembers(Collection<String> groupNames) {
return StreamEx.of(groupNames).mapToEntry(nameToGroup::get)
.nonNullValues().mapValues(Group::getMembers).toMap();
}
```
Pairwise differences:
```java
DoubleStreamEx.of(input).pairMap((a, b) -> b-a).toArray();
```
Support of byte/char/short/float types:
```java
short[] multiply(short[] src, short multiplier) {
return IntStreamEx.of(src).map(x -> x*multiplier).toShortArray();
}
```
Define custom lazy intermediate operation recursively:
```java
static <T> StreamEx<T> scanLeft(StreamEx<T> input, BinaryOperator<T> operator) {
return input.headTail((head, tail) -> scanLeft(tail.mapFirst(cur -> operator.apply(head, cur)), operator)
.prepend(head));
}
```
And more!
### License
This project is licensed under [Apache License, version 2.0](https://www.apache.org/licenses/LICENSE-2.0)
### Installation
Releases are available in [Maven Central](https://repo1.maven.org/maven2/one/util/streamex/)
Before updating StreamEx check the [migration notes](wiki/MIGRATION.md) and full list of [changes](wiki/CHANGES.md).
#### Maven
Add this snippet to the pom.xml `dependencies` section:
```xml
<dependency>
<groupId>one.util</groupId>
<artifactId>streamex</artifactId>
<version>0.8.2</version>
</dependency>
```
#### Gradle
Add this snippet to the build.gradle `dependencies` section:
```groovy
implementation 'one.util:streamex:0.8.2'
```
Pull requests are welcome.
| 0 |
infinilabs/analysis-ik | 🚌 The IK Analysis plugin integrates Lucene IK analyzer into Elasticsearch and OpenSearch, support customized dictionary. | analyzer easysearch elasticsearch ik-analysis java opensearch | IK Analysis for Elasticsearch and OpenSearch
==================================

The IK Analysis plugin integrates Lucene IK analyzer, and support customized dictionary. It supports major versions of Elasticsearch and OpenSearch. Maintained and supported with ❤️ by [INFINI Labs](https://infinilabs.com).
The plugin comprises analyzer: `ik_smart` , `ik_max_word`, and tokenizer: `ik_smart` , `ik_max_word`
# How to Install
You can download the packaged plugins from here: `https://release.infinilabs.com/`,
or you can use the `plugin` cli to install the plugin like this:
For Elasticsearch
```
bin/elasticsearch-plugin install https://get.infini.cloud/elasticsearch/analysis-ik/8.4.1
```
For OpenSearch
```
bin/opensearch-plugin install https://get.infini.cloud/opensearch/analysis-ik/2.12.0
```
Tips: replace your own version number related to your elasticsearch or opensearch.
# Getting Started
1.create a index
```bash
curl -XPUT http://localhost:9200/index
```
2.create a mapping
```bash
curl -XPOST http://localhost:9200/index/_mapping -H 'Content-Type:application/json' -d'
{
"properties": {
"content": {
"type": "text",
"analyzer": "ik_max_word",
"search_analyzer": "ik_smart"
}
}
}'
```
3.index some docs
```bash
curl -XPOST http://localhost:9200/index/_create/1 -H 'Content-Type:application/json' -d'
{"content":"美国留给伊拉克的是个烂摊子吗"}
'
```
```bash
curl -XPOST http://localhost:9200/index/_create/2 -H 'Content-Type:application/json' -d'
{"content":"公安部:各地校车将享最高路权"}
'
```
```bash
curl -XPOST http://localhost:9200/index/_create/3 -H 'Content-Type:application/json' -d'
{"content":"中韩渔警冲突调查:韩警平均每天扣1艘中国渔船"}
'
```
```bash
curl -XPOST http://localhost:9200/index/_create/4 -H 'Content-Type:application/json' -d'
{"content":"中国驻洛杉矶领事馆遭亚裔男子枪击 嫌犯已自首"}
'
```
4.query with highlighting
```bash
curl -XPOST http://localhost:9200/index/_search -H 'Content-Type:application/json' -d'
{
"query" : { "match" : { "content" : "中国" }},
"highlight" : {
"pre_tags" : ["<tag1>", "<tag2>"],
"post_tags" : ["</tag1>", "</tag2>"],
"fields" : {
"content" : {}
}
}
}
'
```
Result
```json
{
"took": 14,
"timed_out": false,
"_shards": {
"total": 5,
"successful": 5,
"failed": 0
},
"hits": {
"total": 2,
"max_score": 2,
"hits": [
{
"_index": "index",
"_type": "fulltext",
"_id": "4",
"_score": 2,
"_source": {
"content": "中国驻洛杉矶领事馆遭亚裔男子枪击 嫌犯已自首"
},
"highlight": {
"content": [
"<tag1>中国</tag1>驻洛杉矶领事馆遭亚裔男子枪击 嫌犯已自首 "
]
}
},
{
"_index": "index",
"_type": "fulltext",
"_id": "3",
"_score": 2,
"_source": {
"content": "中韩渔警冲突调查:韩警平均每天扣1艘中国渔船"
},
"highlight": {
"content": [
"均每天扣1艘<tag1>中国</tag1>渔船 "
]
}
}
]
}
}
```
# Dictionary Configuration
Config file `IKAnalyzer.cfg.xml` can be located at `{conf}/analysis-ik/config/IKAnalyzer.cfg.xml`
or `{plugins}/elasticsearch-analysis-ik-*/config/IKAnalyzer.cfg.xml`
```xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE properties SYSTEM "http://java.sun.com/dtd/properties.dtd">
<properties>
<entry key="ext_dict">custom/mydict.dic;custom/single_word_low_freq.dic</entry>
<entry key="ext_stopwords">custom/ext_stopword.dic</entry>
<entry key="remote_ext_dict">location</entry>
<entry key="remote_ext_stopwords">http://xxx.com/xxx.dic</entry>
</properties>
```
## Hot-reload Dictionary
The current plugin supports hot reloading dictionary for IK Analysis, through the configuration mentioned earlier in the IK configuration file.
```xml
<entry key="remote_ext_dict">location</entry>
<entry key="remote_ext_stopwords">location</entry>
```
Among which `location` refers to a URL, such as `http://yoursite.com/getCustomDict`. This request only needs to meet the following two points to complete the segmentation hot update.
1. The HTTP request needs to return two headers, one is `Last-Modified`, and the other is `ETag`. Both of these are of string type, and if either changes, the plugin will fetch new segmentation to update the word library.
2. The content format returned by the HTTP request is one word per line, and the newline character is represented by `\n`.
Meeting the above two requirements can achieve hot word updates without the need to restart the ES instance.
You can place the hot words that need to be automatically updated in a .txt file encoded in UTF-8. Place it under nginx or another simple HTTP server. When the .txt file is modified, the HTTP server will automatically return the corresponding Last-Modified and ETag when the client requests the file. You can also create a separate tool to extract relevant vocabulary from the business system and update this .txt file.
## FAQs
-------
1. Why isn't the custom dictionary taking effect?
Please ensure that the text format of your custom dictionary is UTF8 encoded.
2. What is the difference between ik_max_word and ik_smart?
ik_max_word: Performs the finest-grained segmentation of the text. For example, it will segment "中华人民共和国国歌" into "中华人民共和国,中华人民,中华,华人,人民共和国,人民,人,民,共和国,共和,和,国国,国歌", exhaustively generating various possible combinations, suitable for Term Query.
ik_smart: Performs the coarsest-grained segmentation of the text. For example, it will segment "中华人民共和国国歌" into "中华人民共和国,国歌", suitable for Phrase queries.
Note: ik_smart is not a subset of ik_max_word.
# Community
Fell free to join the Discord server to discuss anything around this project:
[https://discord.gg/4tKTMkkvVX](https://discord.gg/4tKTMkkvVX)
# License
Copyright ©️ INFINI Labs.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
| 0 |
WeiYe-Jing/datax-web | DataX集成可视化页面,选择数据源即可一键生成数据同步任务,支持RDBMS、Hive、HBase、ClickHouse、MongoDB等数据源,批量创建RDBMS数据同步任务,集成开源调度系统,支持分布式、增量同步数据、实时查看运行日志、监控执行器资源、KILL运行进程、数据源信息加密等。 | null | 




# DataX-Web
[](https://starchart.cc/WeiYe-Jing/datax-web)
DataX Web是在DataX之上开发的分布式数据同步工具,提供简单易用的
操作界面,降低用户使用DataX的学习成本,缩短任务配置时间,避免配置过程中出错。用户可通过页面选择数据源即可创建数据同步任务,支持RDBMS、Hive、HBase、ClickHouse、MongoDB等数据源,RDBMS数据源可批量创建数据同步任务,支持实时查看数据同步进度及日志并提供终止同步功能,集成并二次开发xxl-job可根据时间、自增主键增量同步数据。
任务"执行器"支持集群部署,支持执行器多节点路由策略选择,支持超时控制、失败重试、失败告警、任务依赖,执行器CPU.内存.负载的监控等等。后续还将提供更多的数据源支持、数据转换UDF、表结构同步、数据同步血缘等更为复杂的业务场景。
# Architecture diagram:

# System Requirements
- Language: Java 8(jdk版本建议1.8.201以上)<br>
Python2.7(支持Python3需要修改替换datax/bin下面的三个python文件,替换文件在doc/datax-web/datax-python3下)
- Environment: MacOS, Windows,Linux
- Database: Mysql5.7
# Features
- 1、通过Web构建DataX Json;
- 2、DataX Json保存在数据库中,方便任务的迁移,管理;
- 3、Web实时查看抽取日志,类似Jenkins的日志控制台输出功能;
- 4、DataX运行记录展示,可页面操作停止DataX作业;
- 5、支持DataX定时任务,支持动态修改任务状态、启动/停止任务,以及终止运行中任务,即时生效;
- 6、调度采用中心式设计,支持集群部署;
- 7、任务分布式执行,任务"执行器"支持集群部署;
- 8、执行器会周期性自动注册任务, 调度中心将会自动发现注册的任务并触发执行;
- 9、路由策略:执行器集群部署时提供丰富的路由策略,包括:第一个、最后一个、轮询、随机、一致性HASH、最不经常使用、最近最久未使用、故障转移、忙碌转移等;
- 10、阻塞处理策略:调度过于密集执行器来不及处理时的处理策略,策略包括:单机串行(默认)、丢弃后续调度、覆盖之前调度;
- 11、任务超时控制:支持自定义任务超时时间,任务运行超时将会主动中断任务;
- 12、任务失败重试:支持自定义任务失败重试次数,当任务失败时将会按照预设的失败重试次数主动进行重试;
- 13、任务失败告警;默认提供邮件方式失败告警,同时预留扩展接口,可方便的扩展短信、钉钉等告警方式;
- 14、用户管理:支持在线管理系统用户,存在管理员、普通用户两种角色;
- 15、任务依赖:支持配置子任务依赖,当父任务执行结束且执行成功后将会主动触发一次子任务的执行, 多个子任务用逗号分隔;
- 16、运行报表:支持实时查看运行数据,以及调度报表,如调度日期分布图,调度成功分布图等;
- 17、指定增量字段,配置定时任务自动获取每次的数据区间,任务失败重试,保证数据安全;
- 18、页面可配置DataX启动JVM参数;
- 19、数据源配置成功后添加手动测试功能;
- 20、可以对常用任务进行配置模板,在构建完JSON之后可选择关联模板创建任务;
- 21、jdbc添加hive数据源支持,可在构建JSON页面选择数据源生成column信息并简化配置;
- 22、优先通过环境变量获取DataX文件目录,集群部署时不用指定JSON及日志目录;
- 23、通过动态参数配置指定hive分区,也可以配合增量实现增量数据动态插入分区;
- 24、任务类型由原来DataX任务扩展到Shell任务、Python任务、PowerShell任务;
- 25、添加HBase数据源支持,JSON构建可通过HBase数据源获取hbaseConfig,column;
- 26、添加MongoDB数据源支持,用户仅需要选择collectionName即可完成json构建;
- 27、添加执行器CPU、内存、负载的监控页面;
- 28、添加24类插件DataX JSON配置样例
- 29、公共字段(创建时间,创建人,修改时间,修改者)插入或更新时自动填充
- 30、对swagger接口进行token验证
- 31、任务增加超时时间,对超时任务kill datax进程,可配合重试策略避免网络问题导致的datax卡死。
- 32、添加项目管理模块,可对任务分类管理;
- 33、对RDBMS数据源增加批量任务创建功能,选择数据源,表即可根据模板批量生成DataX同步任务;
- 34、JSON构建增加ClickHouse数据源支持;
- 35、执行器CPU.内存.负载的监控页面图形化;
- 36、RDBMS数据源增量抽取增加主键自增方式并优化页面参数配置;
- 37、更换MongoDB数据源连接方式,重构HBase数据源JSON构建模块;
- 38、脚本类型任务增加停止功能;
- 39、rdbms json构建增加postSql,并支持构建多个preSql,postSql;
- 40、数据源信息加密算法修改及代码优化;
- 41、日志页面增加DataX执行结果统计数据;
# Quick Start:
##### 请点击:[Quick Start](https://github.com/WeiYe-Jing/datax-web/blob/master/userGuid.md)
##### Linux:[一键部署](https://github.com/WeiYe-Jing/datax-web/blob/master/doc/datax-web/datax-web-deploy.md)
# Introduction:
### 1.执行器配置(使用开源项目xxl-job)

- 1、"调度中心OnLine:"右侧显示在线的"调度中心"列表, 任务执行结束后, 将会以failover的模式进行回调调度中心通知执行结果, 避免回调的单点风险;
- 2、"执行器列表" 中显示在线的执行器列表, 可通过"OnLine 机器"查看对应执行器的集群机器;
#### 执行器属性说明

```
1、AppName: (与datax-executor中application.yml的datax.job.executor.appname保持一致)
每个执行器集群的唯一标示AppName, 执行器会周期性以AppName为对象进行自动注册。可通过该配置自动发现注册成功的执行器, 供任务调度时使用;
2、名称: 执行器的名称, 因为AppName限制字母数字等组成,可读性不强, 名称为了提高执行器的可读性;
3、排序: 执行器的排序, 系统中需要执行器的地方,如任务新增, 将会按照该排序读取可用的执行器列表;
4、注册方式:调度中心获取执行器地址的方式;
自动注册:执行器自动进行执行器注册,调度中心通过底层注册表可以动态发现执行器机器地址;
手动录入:人工手动录入执行器的地址信息,多地址逗号分隔,供调度中心使用;
5、机器地址:"注册方式"为"手动录入"时有效,支持人工维护执行器的地址信息;
```
### 2.创建数据源

第四步使用
### 3.创建任务模版

第四步使用
### 4. 构建JSON脚本
- 1.步骤一,步骤二,选择第二步中创建的数据源,JSON构建目前支持的数据源有hive,mysql,oracle,postgresql,sqlserver,hbase,mongodb,clickhouse 其它数据源的JSON构建正在开发中,暂时需要手动编写。

- 2.字段映射

- 3.点击构建,生成json,此时可以选择复制json然后创建任务,选择datax任务,将json粘贴到文本框。也可以点击选择模版,直接生成任务。

### 5.批量创建任务


### 6.任务创建介绍(关联模版创建任务不再介绍,具体参考4. 构建JSON脚本)
#### 支持DataX任务,Shell任务,Python任务,PowerShell任务


- 阻塞处理策略:调度过于密集执行器来不及处理时的处理策略;
- 单机串行:调度请求进入单机执行器后,调度请求进入FIFO队列并以串行方式运行;
- 丢弃后续调度:调度请求进入单机执行器后,发现执行器存在运行的调度任务,本次请求将会被丢弃并标记为失败;
- 覆盖之前调度:调度请求进入单机执行器后,发现执行器存在运行的调度任务,将会终止运行中的调度任务并清空队列,然后运行本地调度任务;
- 增量增新建议将阻塞策略设置为丢弃后续调度或者单机串行
- 设置单机串行时应该注意合理设置重试次数(失败重试的次数*每次执行时间<任务的调度周期),重试的次数如果设置的过多会导致数据重复,例如任务30秒执行一次,每次执行时间需要20秒,设置重试三次,如果任务失败了,第一个重试的时间段为1577755680-1577756680,重试任务没结束,新任务又开启,那新任务的时间段会是1577755680-1577758680
- [增量参数设置](https://github.com/WeiYe-Jing/datax-web/blob/master/doc/datax-web/increment-desc.md)
- [分区参数设置](https://github.com/WeiYe-Jing/datax-web/blob/master/doc/datax-web/partition-dynamic-param.md)
### 7. 任务列表

### 8. 可以点击查看日志,实时获取日志信息,终止正在执行的datax进程


### 9.任务资源监控

### 10. admin可以创建用户,编辑用户信息

# UI
[前端github地址](https://github.com/WeiYe-Jing/datax-web-ui)
# 项目成员
- water
```
非常荣幸成为datax-web的Committer,从早期datax手工编写任务+配置,到datax-web界面化勾选创建任务+配置信息+调度管理,datax-web将数据同步工作的效率提升不少,相信后面后成为etl中不可或缺的生产力……
```
- Alecor
```
非常荣幸成为datax-web的Committer,datax-web旨在帮助用户从datax配置中解放出来,提供datax的Web化的管理能力。希望datax-web能为更多有需要的人服务,带来更好的简单、易用的体验!
```
- zhouhongfa
- liukunyuan
感谢贡献!
# Contributing
Contributions are welcome! Open a pull request to fix a bug, or open an Issue to discuss a new feature or change.
欢迎参与项目贡献!比如提交PR修复一个bug,或者新建 Issue 讨论新特性或者变更。
# Copyright and License
MIT License
Copyright (c) 2020 WeiYe
产品开源免费,并且将持续提供免费的社区技术支持。个人或企业内部可自由的接入和使用。
> 欢迎在 [登记地址](https://github.com/WeiYe-Jing/datax-web/issues/93) 登记,登记仅仅为了产品推广和提升社区开发的动力。
# v-2.1.2
### 新增
1. 添加项目管理模块,可对任务分类管理;
2. 对RDBMS数据源增加批量任务创建功能,选择数据源,表即可根据模板批量生成DataX同步任务;
3. JSON构建增加ClickHouse数据源支持;
4. 执行器CPU.内存.负载的监控页面图形化;
5. RDBMS数据源增量抽取增加主键自增方式并优化页面参数配置;
6. 更换MongoDB数据源连接方式,重构HBase数据源JSON构建模块;
7. 脚本类型任务增加停止功能;
8. rdbms json构建增加postSql,并支持构建多个preSql,postSql;
9. 合并datax-registry模块到datax-rpc中;
10.数据源信息加密算法修改及代码优化;
11.时间增量同步支持更多时间格式;
12.日志页面增加DataX执行结果统计数据;
### 升级:
1. PostgreSql,SQLServer,Oracle 数据源JSON构建增加schema name选择;
2. DataX JSON中的字段名称与数据源关键词一致问题优化;
3. 任务管理页面按钮展示优化;
4. 日志管理页面增加任务描述信息;
5. JSON构建前端form表单不能缓存数据问题修复;
6. HIVE JSON构建增加头尾选项参数;
### 备注:
2.1.1版本不建议升级,数据源信息加密方式变更会导致之前已加密的数据源解密失败,任务运行失败。
如果需要升级请重建数据源,任务。
# v-2.1.1
### 新增
1. 添加HBase数据源支持,JSON构建可通过HBase数据源获取hbaseConfig,column;
2. 添加MongoDB数据源支持,用户仅需要选择collectionName即可完成json构建;
3. 添加执行器CPU.内存.负载的监控页面;
4. 添加24类插件DataX JSON配置样例
5. 公共字段(创建时间,创建人,修改时间,修改者)插入或更新时自动填充
6. 对swagger接口进行token验证
7. 任务增加超时时间,对超时任务kill datax进程,可配合重试策略避免网络问题导致的datax卡死。
### 升级:
1. 数据源管理对用户名和密码进行加密,提高安全性;
2. 对JSON文件中的用户名密码进行加密,执行DataX任务时解密
3. 对页面菜单整理,图标升级,提示信息等交互优化;
4. 日志输出取消项目类名等无关信息,减小文件大小,优化大文件输出,优化页面展示;
5. logback为从yml中获取日志路径配置
### 修复:
1. 任务日志过大时,查看日志报错,请求超时;
# 提交代码
[参与贡献](https://github.com/WeiYe-Jing/datax-web/issues/190)
# Contact us
### 个人微信

### QQ交流群

| 0 |
zfoo-project/zfoo | 💡Extremely fast enterprise server framework, can be used in RPC, game server, web server. | byte-buddy cocos cpp game-framework game-server godot godot-engine hotswap java javassist mongodb netty network orm rpc serialization spring unity unreal-engine websocket | English | [简体中文](./README_CN.md)
<a href="https://github.com/zfoo-project/zfoo"><img src="/doc/image/logo.jpg" width="30%"></a>
## Ⅰ. Introduction of zfoo🚩
- **Extremely fast, asynchronous, actor design, lock free, universal RPC framework, native GraalVM support**
- **Decentralized serialization [zfoo protocol](protocol/README.md)**, supports C++ C# Go Java Javascript TypeScript Lua GDScript Python
- **High scalability**, Single server deployment, microservice deployment, cluster deployment, gateway deployment
- **Can be used as a game server framework or website server framework.**
Perfect work development process, complete online solution
- Spring projects, distributed projects, container projects, **hot update code without
downtime** [hotswap](hotswap/src/test/java/com/zfoo/hotswap/ApplicationTest.java)
- Excel json csv configuration is automatically mapped and parsed, **Online hotswap
configuration** [storage](storage/src/test/java/com/zfoo/storage/ApplicationTest.java)
- Automapping framework for MongoDB [orm](orm/README.md)
- Event bus [event](event/src/test/java/com/zfoo/event/ApplicationTest.java)
- Time task scheduling [scheduler](scheduler/README.md)
- **Cpu, memory, hard disk, network monitoring built into the program** no code and extra tools
required [monitor](monitor/src/test/java/com/zfoo/monitor/ApplicationTest.java)
## Ⅱ. Who uses this project
- Projects with extremely high-performance requirements, such as website and game server frameworks, single server, global server, live chat, IM system, real-time push
- Projects such as saving, development, deployment, operation and maintenance costs
- As a backend infrastructure for **Godot, Unity, Cocos,Webgl, H5**, Network protocol supports tcp udp websocket http
- [Keep it Simple and Stupid](https://baike.baidu.com/item/KISS原则/3242383), simple configuration, lightweight code
## Ⅲ. Maven dependency✨
- Environment requirement **JDK 17+**, support **OpenJDK**, **Oracle JDK** and **native GraalVM**
```
<dependency>
<groupId>com.zfoo</groupId>
<artifactId>boot</artifactId>
<version>3.3.1</version>
</dependency>
```
- If you don't want to depend on all zfoo modules, you only need to choose to depend on one of them
```
<dependency>
<groupId>com.zfoo</groupId>
<artifactId>protocol</artifactId>
<version>3.3.1</version>
</dependency>
```
## Ⅳ. Tutorials
- [zfoo sdk of csharp and lua for unity and godot](https://github.com/zfoo-project/zfoo-sdk-csharp-lua-unity-godot)
- [zfoo sdk typescript javascript cocos web h5](https://github.com/zfoo-project/zfoo-sdk-typescript-javascript-cocos-web-h5)
- [zfoo sdk gdscript for godot](https://github.com/zfoo-project/zfoo-sdk-gdscript-godot)
- [tank-game-server](https://github.com/zfoo-project/tank-game-server) Online game《The Fight of Tanks》, novice friendly,
difficulty 2 stars
- [godot-bird](https://github.com/zfoo-project/godot-bird) bird and bird,powered by godot
- [FAQ](./doc/FAQ.md),There are standard demo display and instructions in the test folder of each project directory,
which can be run directly
## Ⅴ. Usage⭐
#### 1. [protocol](protocol/README.md) ultimate performance serialization and deserialization
```
// zfoo protocol registration, can only be initialized once
ProtocolManager.initProtocol(Set.of(ComplexObject.class, ObjectA.class, ObjectB.class));
// serialization
ProtocolManager.write(byteBuf, complexObject);
// deserialization
var packet = ProtocolManager.read(byteBuf);
```
#### 2. [net](net/README.md) ultimate performance RPC framework, supports tcp udp websocket http
```
// Service provider, only need to add an annotation to the method, the interface will be automatically registered
@PacketReceiver
public void atUserInfoAsk(Session session, UserInfoAsk ask) {
}
// Consumers, synchronously requesting remote service, will block the current thread
var userInfoAsk = UserInfoAsk.valueOf(userId);
var answer = NetContext.getCosumer().syncAsk(userInfoAsk, UserInfoAnswer.class, userId).packet();
// Consumers, asynchronously requesting remote service, and will still execute logic in the current thread after the asynchronous
NetContext.getCosumer()
.asyncAsk(userInfoAsk, UserInfoAnswer.class, userId)
.whenComplete(sm -> {
// do something
);
```
#### 3. [hotswap](hotswap/src/test/java/com/zfoo/hotswap/ApplicationTest.java) hot update code, no need to stop the server, no additional configuration, just one line of code to start hot update
```
// Pass in the class file that needs to be updated
HotSwapUtils.hotswapClass(bytes);
```
#### 4. [orm](orm/README.md) automatic mapping framework based on mongodb
```
// You don't need to write sql and any configuration yourself, define a table in the database directly through annotation definitions
@EntityCache
public class UserEntity implements IEntity<Long> {
@Id
private long id;
private String name;
}
// update database data
entityCaches.update(userEntity);
```
#### 5. [event](event/src/test/java/com/zfoo/event/ApplicationTest.java) use the observer design pattern, decouples different modules and improves the quality of the code
```
// To receive an event, you only need to add an annotation to the method and the method will be automatically listen for the event
@EventReceiver
public void onMyNoticeEvent(MyNoticeEvent event) {
// do something
}
// fire an event
EventBus.post(MyNoticeEvent.valueOf("My event"));
```
#### 6. [scheduler](scheduler/README.md) scheduling Framework Based on Cron Expression
````
@Scheduler(cron = "0/1 * * * * ?")
public void cronSchedulerPerSecond() {
// do something
}
````
#### 7. [storage](storage/src/test/java/com/zfoo/storage/ApplicationTest.java) Excel to class automatic mapping framework, you only need to define a class corresponding to Excel, and directly parse Excel
```
@Storage
public class StudentResource {
@Id
private int id;
@Index
private String name;
private int age;
}
```
## Ⅵ. Commit specification👏
- People who like this project are welcome to maintain this project together, and pay attention to the following
specifications when submitting code
- The code formats use the default formatting of IntelliJ Idea
- [conventional-changelog-metahub](https://github.com/pvdlg/conventional-changelog-metahub#commit-types)
## Ⅶ. License
zfoo use [Apache License Version 2.0](http://www.apache.org/licenses/LICENSE-2.0)

| 0 |
careercup/ctci | Cracking the Coding Interview, 5th Edition | null | ctci
====
Solutions for "Cracking the Coding Interview v5"
Adding equivalent solutions in Objective-C
Adding my own solutions
| 0 |
M66B/NetGuard | A simple way to block access to the internet per app | null | # NetGuard
*NetGuard* provides simple and advanced ways to block access to the internet - no root required.
Applications and addresses can individually be allowed or denied access to your Wi-Fi and/or mobile connection.
<br>
**WARNING: there is an app in the Samsung Galaxy app store "*Play Music - MP3 Music player*"
with the same package name as NetGuard, which will be installed as update without your confirmation.
This app is probably malicious and was reported to Samsung on December 8, 2021.**
<br>
Blocking access to the internet can help:
* reduce your data usage
* save your battery
* increase your privacy
NetGuard is the first free and open source no-root firewall for Android.
Features:
* Simple to use
* No root required
* 100% open source
* No calling home
* No tracking or analytics
* Actively developed and supported
* Android 5.1 and later supported
* IPv4/IPv6 TCP/UDP supported
* Tethering supported
* Optionally allow when screen on
* Optionally block when roaming
* Optionally block system applications
* Optionally forward ports, also to external addresses (not available if installed from the Play store)
* Optionally notify when an application accesses the internet
* Optionally record network usage per application per address
* Optionally [block ads using a hosts file](https://github.com/M66B/NetGuard/blob/master/ADBLOCKING.md) (not available if installed from the Play store)
* Material design theme with light and dark theme
PRO features:
* Log all outgoing traffic; search and filter access attempts; export PCAP files to analyze traffic
* Allow/block individual addresses per application
* New application notifications; configure NetGuard directly from the notification
* Display network speed graph in a status bar notification
* Select from five additional themes in both light and dark version
There is no other no-root firewall offering all these features.
Requirements:
* Android 5.1 or later
* A [compatible device](#compatibility)
Downloads:
* [GitHub](https://github.com/M66B/NetGuard/releases)
* [Google Play](https://play.google.com/store/apps/details?id=eu.faircode.netguard)
Certificate fingerprints:
* MD5: B6:4A:E8:08:1C:3C:9C:19:D6:9E:29:00:46:89:DA:73
* SHA1: EF:46:F8:13:D2:C8:A0:64:D7:2C:93:6B:9B:96:D1:CC:CC:98:93:78
* SHA256: E4:A2:60:A2:DC:E7:B7:AF:23:EE:91:9C:48:9E:15:FD:01:02:B9:3F:9E:7C:9D:82:B0:9C:0B:39:50:00:E4:D4
Usage:
* Enable the firewall using the switch in the action bar
* Allow/deny Wi-Fi/mobile internet access using the icons along the right side of the application list
You can use the settings menu to change from blacklist mode (allow all in *Settings* but block unwanted applications in list) to whitelist mode (block all in *Settings* but allow favorite applications in list).
* Red/orange/yellow/amber = internet access denied
* Teal/blue/purple/grey = internet access allowed
<img src="https://raw.githubusercontent.com/M66B/NetGuard/master/screenshots/01-main.png" width="320" height="569" />
<img src="https://raw.githubusercontent.com/M66B/NetGuard/master/screenshots/02-main-details.png" width="320" height="569" />
<img src="https://raw.githubusercontent.com/M66B/NetGuard/master/screenshots/03-main-access.png" width="320" height="569" />
<img src="https://raw.githubusercontent.com/M66B/NetGuard/master/screenshots/08-notifications.png" width="320" height="569" />
For more screenshots, see [here](https://github.com/M66B/NetGuard/tree/master/screenshots).
Compatibility
-------------
The only way to build a no-root firewall on Android is to use the Android VPN service.
Android doesn't allow chaining of VPN services, so you cannot use NetGuard together with other VPN based applications.
See also [this FAQ](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq2).
NetGuard can be used on rooted devices too and even offers more features than most root firewalls.
Some older Android versions, especially Samsung's Android versions, have a buggy VPN implementation,
which results in Android refusing to start the VPN service in certain circumstances,
like when there is no internet connectivity yet (when starting up your device)
or when incorrectly requiring manual approval of the VPN service again (when starting up your device).
NetGuard will try to workaround this and remove the error message when it succeeds, else you are out of luck.
Some LineageOS versions have a broken Android VPN implementation, causing all traffic to be blocked,
please see [this FAQ](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq51) for more information.
On GrapheneOS, the Android *Always-On VPN* function and the sub option '*Block connections without VPN*' are enabled by default.
However, this sub option will result in blocking all traffic, please see [this FAQ](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq51).
NetGuard is not supported for apps installed in a [work profile](https://developer.android.com/work/managed-profiles),
or in a [Secure Folder](https://www.samsung.com/uk/support/mobile-devices/what-is-the-secure-folder-and-how-do-i-use-it/) (Samsung),
or as second instance (MIUI), or as Parallel app (OnePlus), or as Xiaomi dual app
because the Android VPN service too often does not work correctly in this situation, which can't be fixed by NetGuard.
NetGuard is not supported for internet connections via a wire, like ethernet or USB,
because the Android VPN service often doesn't work properly in this situation.
Filtering mode cannot be used on [CopperheadOS](https://copperhead.co/android/).
NetGuard will not work or crash when the package *com.android.vpndialogs* has been removed or otherwise is unavailable.
Removing this package is possible with root permissions only.
If you disable this package, you can enable it with this command again:
```
adb shell pm enable --user 0 com.android.vpndialogs
```
NetGuard is supported on phones and tablets with a true-color screen only, so not for other device types like on a television or in a car.
Android does not allow incoming connections (not the same as incoming traffic) and the Android VPN service has no support for this either.
Therefore managing incoming connections for servers running on your device is not supported.
Wi-Fi or IP calling will not work if your provider uses [IPsec](https://en.wikipedia.org/wiki/IPsec) to encrypt your phone calls, SMS messages and/or MMS messages,
unless there was made an exception in NetGuard for your provider (currently for T-Mobile and Verizon).
I am happy to add exceptions for other providers, but I need the [MCC](https://en.wikipedia.org/wiki/Mobile_country_code) codes, [MNC](https://en.wikipedia.org/wiki/MNC) codes and [IP address](https://en.wikipedia.org/wiki/IP_address) ranges your provider is using.
As an alternative you can enable the option '*Disable on call*', which is available since version 2.113.
<a name="FAQ"></a>
Frequently Asked Questions (FAQ)
--------------------------------
<a name="FAQ0"></a>
[**(0) How do I use NetGuard?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq0)
<a name="FAQ1"></a>
[**(1) Can NetGuard completely protect my privacy?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq1)
<a name="FAQ2"></a>
[**(2) Can I use another VPN application while using NetGuard?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq2)
<a name="FAQ3"></a>
[**(3) Can I use NetGuard on any Android version?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq3)
<a name="FAQ4"></a>
[**(4) Will NetGuard use extra battery power?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq4)
<a name="FAQ6"></a>
[**(6) Will NetGuard send my internet traffic to an external (VPN) server?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq6)
<a name="FAQ7"></a>
[**(7) Why are applications without internet permission shown?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq7)
<a name="FAQ8"></a>
[**(8) What do I need to enable for the Google Play™ store app to work?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq8)
<a name="FAQ9"></a>
[**(9) Why is the VPN service being restarted?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq9)
<a name="FAQ10"></a>
[**(10) Will you provide a Tasker plug-in?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq10)
<a name="FAQ13"></a>
[**(13) How can I remove the ongoing NetGuard entry in the notification screen?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq13)
<a name="FAQ14"></a>
[**(14) Why can't I select OK to approve the VPN connection request?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq14)
<a name="FAQ15"></a>
[**(15) Are F-Droid builds supported?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq15)
<a name="FAQ16"></a>
[**(16) Why are some applications shown dimmed?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq16)
<a name="FAQ17"></a>
[**(17) Why is NetGuard using so much memory?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq17)
<a name="FAQ18"></a>
[**(18) Why can't I find NetGuard in the Google Play™ store app?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq18)
<a name="FAQ19"></a>
[**(19) Why does application XYZ still have internet access?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq19)
<a name="FAQ20"></a>
[**(20) Can I Greenify/hibernate NetGuard?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq20)
<a name="FAQ21"></a>
[**(21) Does doze mode affect NetGuard?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq21)
<a name="FAQ22"></a>
[**(22) Can I tether (use the Android hotspot) / use Wi-Fi calling while using NetGuard?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq22)
<a name="FAQ24"></a>
[**(24) Can you remove the notification from the status bar?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq24)
<a name="FAQ25"></a>
[**(25) Can you add a 'select all'?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq25)
<a name="FAQ27"></a>
[**(27) How do I read the blocked traffic log?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq27)
<a name="FAQ28"></a>
[**(28) Why is Google connectivity services allowed internet access by default?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq28)
<a name="FAQ29"></a>
[**(29) Why do I get 'The item you requested is not available for purchase'?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq29)
<a name="FAQ30"></a>
[**(30) Can I also run AFWall+ on the same device?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq30)
<a name="FAQ31"></a>
[**(31) Why can some applications be configured as a group only?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq31)
<a name="FAQ32"></a>
[**(32) Why is the battery/network usage of NetGuard so high**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq32)
<a name="FAQ33"></a>
[**(33) Can you add profiles?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq33)
<a name="FAQ34"></a>
[**(34) Can you add the condition 'when on foreground'?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq34)
<a name="FAQ35"></a>
[**(35) Why does the VPN not start?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq35)
<a name="FAQ36"></a>
[**(36) Can you add PIN or password protection?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq36)
<a name="FAQ37"></a>
[**(37) Why are the pro features so expensive?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq37)
<a name="FAQ38"></a>
[**(38) Why did NetGuard stop running?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq38)
<a name="FAQ39"></a>
[**(39) How does a VPN based firewall differ from a iptables based firewall?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq39)
<a name="FAQ40"></a>
[**(40) Can you add schedules?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq40)
<a name="FAQ41"></a>
[**(41) Can you add wildcards?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq41)
<a name="FAQ42"></a>
[**(42) Why is permission ... needed?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq42)
<a name="FAQ43"></a>
[**(43) I get 'This app is causing your device to run slowly'**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq43)
<a name="FAQ44"></a>
[**(44) I don't get notifications on access**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq44)
<a name="FAQ45"></a>
[**(45) Does NetGuard handle incoming connections?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq45)
<a name="FAQ46"></a>
[**(46) Can I get a refund?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq46)
<a name="FAQ47"></a>
[**(47) Why are there in application advertisements?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq47)
<a name="FAQ48"></a>
[**(48) Why are some domain names blocked while they are set to be allowed?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq48)
<a name="FAQ49"></a>
[**(49) Does NetGuard encrypt my internet traffic / hide my IP address?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq49)
<a name="FAQ50"></a>
[**(50) Will NetGuard automatically start on boot?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq50)
<a name="FAQ51"></a>
[**(51) NetGuard blocks all internet traffic!**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq51)
<a name="FAQ52"></a>
[**(52) What is lockdown mode?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq52)
<a name="FAQ53"></a>
[**(53) The translation in my language is missing / incorrect / incomplete!**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq53)
<a name="FAQ54"></a>
[**(54) How to tunnel all TCP connections through the Tor network?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq54)
<a name="FAQ55"></a>
[**(55) Why does NetGuard connect to Amazon / ipinfo.io?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq55)
<a name="FAQ56"></a>
[**(56) NetGuard allows all internet traffic!**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq56)
<a name="FAQ57"></a>
[**(57) Why does NetGuard use so much data?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq57)
<a name="FAQ58"></a>
[**(58) Why does loading the application list take a long time?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq58)
<a name="FAQ59"></a>
[**(59) Can you help me restore my purchase?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq59)
<a name="FAQ60"></a>
[**(60) Why does IP (Wi-Fi) calling/SMS/MMS not work?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq60)
<a name="FAQ61"></a>
[**(61) Help, NetGuard crashed!**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq61)
<a name="FAQ62"></a>
[**(62) How can I solve 'There was a problem parsing the package' ?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq62)
<a name="FAQ63"></a>
[**(63) Why is all DNS traffic allowed?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq63)
<a name="FAQ64"></a>
[**(64) Can you add DNS over TLS?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq64)
<a name="FAQ65"></a>
[**(65) Why can NetGuard not block itself?**](https://github.com/M66B/NetGuard/blob/master/FAQ.md#user-content-faq65)
Support
-------
For questions, feature requests and bug reports, please [use this form](https://contact.faircode.eu/?product=netguard%2B).
There is support on the latest version of NetGuard only.
There is no support on things that are not directly related to NetGuard.
There is no support on building and developing things by yourself.
**NetGuard is supported for phones and tablets only, so not for other device types like on a television or in a car.**
Contributing
------------
*Building*
Building is simple, if you install the right tools:
* [Android Studio](http://developer.android.com/sdk/)
* [Android NDK](http://developer.android.com/tools/sdk/ndk/)
The native code is built as part of the Android Studio project.
It is expected that you can solve build problems yourself, so there is no support on building.
If you cannot build yourself, there are prebuilt versions of NetGuard available [here](https://github.com/M66B/NetGuard/releases).
*Translating*
* Translations to other languages are welcomed
* You can translate online [here](https://crowdin.com/project/netguard/)
* If your language is not listed, please send a message to marcel(plus)netguard(at)faircode(dot)eu
* You can see the status of all translations [here](https://crowdin.com/project/netguard).
Please note that by contributing you agree to the license below, including the copyright, without any additional terms or conditions.
Attribution
-----------
NetGuard uses:
* [Glide](https://bumptech.github.io/glide/)
* [Android Support Library](https://developer.android.com/tools/support-library/)
License
-------
[GNU General Public License version 3](http://www.gnu.org/licenses/gpl.txt)
Copyright (c) 2015-2018 Marcel Bokhorst ([M66B](https://contact.faircode.eu/))
All rights reserved
This file is part of NetGuard.
NetGuard is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your discretion) any later version.
NetGuard is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with NetGuard. If not, see [http://www.gnu.org/licenses/](http://www.gnu.org/licenses/).
Trademarks
----------
*Android is a trademark of Google Inc. Google Play is a trademark of Google Inc*
| 0 |
ZHENFENG13/spring-boot-projects | :fire: 该仓库中主要是 Spring Boot 的入门学习教程以及一些常用的 Spring Boot 实战项目教程,包括 Spring Boot 使用的各种示例代码,同时也包括一些实战项目的项目源码和效果展示,实战项目包括基本的 web 开发以及目前大家普遍使用的线上博客项目/企业大型商城系统/前后端分离实践项目等,摆脱各种 hello world 入门案例的束缚,真正的掌握 Spring Boot 开发。 | java microservice mybatis redis spring spring-boot spring-boot-3 spring-boot-demo spring-boot-examples spring-boot-project spring-boot-thymeleaf spring-cloud spring-cloud-alibaba spring6 springboot springboot-demo springboot3 swagger vue vue3 | # Spring Boot Projects
该仓库中主要是 Spring Boot 的入门学习教程以及一些常用的 Spring Boot 实战项目教程,包括 Spring Boot 使用的各种示例代码,同时也包括一些实战项目的项目源码和效果展示,实战项目包括基本的 Web 开发以及目前大家普遍使用的前后端分离实践项目等,后续会根据大家的反馈继续增加一些实战项目源码,摆脱各种 Hello World 入门案例的束缚,真正的掌握 Spring Boot 开发。
实战项目源码都已升级至 Spring Boot 3.x 版本,本仓库中的 Spring Boot 入门案例也已经升级至 Spring Boot 3.x 版本,Java 最低版本要求为 17,请知悉,想要学习和使用 Spring Boot 2.x 版本请自行切换分支。。
## 项目导航
[Spring Boot 入门小案例](./SpringBoot入门案例源码) | [Spring Boot 入门小案例](./SpringBoot入门案例源码) | [Spring Boot 整合案例系列2](./玩转SpringBoot系列案例源码) | [Spring Boot 前后端分离项目实践](./SpringBoot前后端分离实战项目源码) | [Spring Boot 咨询发布系统项目实践](./SpringBoot咨询发布系统实战项目源码) | [Spring Boot + Mybatis + Thymeleaf 实现的开源博客系统](https://github.com/ZHENFENG13/My-Blog) | [Spring Boot + Mybatis + Thymeleaf 实现的 BBS 论坛系统](https://github.com/ZHENFENG13/My-BBS) | [Spring Boot + Mybatis + Thymeleaf 仿知乎专栏项目](https://github.com/ZHENFENG13/My-Column) | [Spring Boot + layui 实现的后台管理系统](https://github.com/ZHENFENG13/My-Blog-layui) | [Spring Boot 大型商城项目实践](https://github.com/newbee-ltd/newbee-mall) | [Spring Boot + Vue 前后端分离商城项目](https://github.com/newbee-ltd/newbee-mall-vue-app) | [Spring Boot + Vue3 前后端分离商城项目](https://github.com/newbee-ltd/newbee-mall-vue3-app) | [Github地址](https://github.com/ZHENFENG13/spring-boot-projects) | [码云 Gitee 地址](https://gitee.com/zhenfeng13/spring-boot-projects)
---
#### 项目演示
- [Spring Boot 前后端分离项目预览](https://www.bilibili.com/video/av52551579)
- [Spring Boot 咨询发布系统项目预览](https://www.bilibili.com/video/av52551450)
- [Spring Boot 开源博客系统预览](https://www.bilibili.com/video/av52551095)
- [Spring Boot 商城项目总览](https://edu.csdn.net/course/play/26258/326466)
- [Spring Boot 商城系统介绍](https://edu.csdn.net/course/play/26258/326467)
- [Spring Boot 商城后台管理系统介绍](https://edu.csdn.net/course/play/26258/328801)
---
#### Spring Boot 实战项目源码
- [前后端分离项目](./SpringBoot前后端分离实战项目源码/spring-boot-project-front-end&back-end)
- [咨询发布项目](./SpringBoot咨询发布系统实战项目源码/springboot-project-news-publish-system)
- [博客系统](https://github.com/ZHENFENG13/My-Blog)
- [BBS 论坛系统](https://github.com/ZHENFENG13/My-BBS)
- [仿知乎专栏项目](https://github.com/ZHENFENG13/My-Column)
- [Spring Boot + layui 实现的后台管理系统](https://github.com/ZHENFENG13/My-Blog-layui)
- [Spring Boot 大型商城项目](https://github.com/newbee-ltd/newbee-mall)
- [Spring Boot + Vue 前后端分离商城项目](https://github.com/newbee-ltd/newbee-mall-vue-app)
- [Spring Boot + Vue3 前后端分离商城项目](https://github.com/newbee-ltd/newbee-mall-vue3-app)
---
## Spring Boot 基础使用案例
**示例代码**
- [spring-boot-hello](./SpringBoot入门案例源码/spring-boot-helloworld):Spring Boot 第一个案例代码
- [spring-boot-logging](./玩转SpringBoot系列案例源码/spring-boot-logging):Spring Boot 基础开发之日志输出案例
- [spring-boot-test](./玩转SpringBoot系列案例源码/spring-boot-test):Spring Boot 基础开发之单元测试案例
- [spring-boot-static-resources](./SpringBoot入门案例源码/spring-boot-static-resources):Spring Boot 基础开发之静态资源处理案例
- [spring-boot-ajax](./SpringBoot入门案例源码/spring-boot-ajax):Spring Boot 项目实践之 Ajax 技术使用教程
- [spring-boot-message-converter](./玩转SpringBoot系列案例源码/spring-boot-message-converter):Spring Boot 基础开发之消息转换器案例
- [spring-boot-jdbc](./SpringBoot入门案例源码/spring-boot-jdbc):Spring Boot 连接数据库以及在 Spring Boot 项目中操作数据库
- [spring-boot-druid](./玩转SpringBoot系列案例源码/spring-boot-druid):Spring Boot 中使用自定义 Druid 数据源案例
- [spring-boot-mybatis](./SpringBoot入门案例源码/spring-boot-mybatis):Spring Boot 整合 MyBatis 操作数据库案例
- [spring-boot-transaction](./玩转SpringBoot系列案例源码/spring-boot-transaction):Spring Boot 中的事务处理案例
- [spring-boot-redis](./玩转SpringBoot系列案例源码/spring-boot-redis):Spring Boot 整合 Redis 操作缓存模块
- [spring-boot-schedule](./玩转SpringBoot系列案例源码/spring-boot-schedule):Spring Boot 项目开发之@Scheduled实现定时任务案例
- [spring-boot-quartz](./玩转SpringBoot系列案例源码/spring-boot-quartz):Spring Boot 项目开发之Quartz定时任务案例
- [spring-boot-error-page](./玩转SpringBoot系列案例源码/spring-boot-error-page):Spring Boot 自定义错误页面案例
- [spring-boot-swagger](./玩转SpringBoot系列案例源码/spring-boot-swagger):Spring Boot 集成 Swagger 生成接口文档案例
- [spring-boot-file-upload](./SpringBoot入门案例源码/spring-boot-file-upload):Spring Boot 处理文件上传及路径回显
- [spring-boot-RESTful-api](./SpringBoot入门案例源码/spring-boot-RESTful-api) :Spring Boot 项目实践之 RESTful API 设计与实现
- [spring-boot-web-jsp](./玩转SpringBoot系列案例源码/spring-boot-web-jsp) :Spring Boot 整合 JSP 开发 web 项目
- [spring-boot-web-thymeleaf](./玩转SpringBoot系列案例源码/spring-boot-web-thymeleaf) :Spring Boot 整合 Thymeleaf 案例
- [spring-boot-web-thymeleaf-syntax](./玩转SpringBoot系列案例源码/spring-boot-web-thymeleaf-syntax) :Thymeleaf 语法详解
- [spring-boot-web-freemarker](./玩转SpringBoot系列案例源码/spring-boot-web-freemarker) :Spring Boot 整合 FreeMarker 案例
- [spring-boot-admin](./玩转SpringBoot系列案例源码/spring-boot-admin) :Spring Boot Admin 整合使用案例
这些案例主要是为了让大家能够掌握 Spring Boot 的开发和使用,旨在让读者更加熟悉 Spring Boot 及企业开发中需要注意的事项并具有使用 Spring Boot 技术进行大部分企业项目功能开发的能力。
关注公众号:**程序员十三**,回复"勾搭"进群交流。
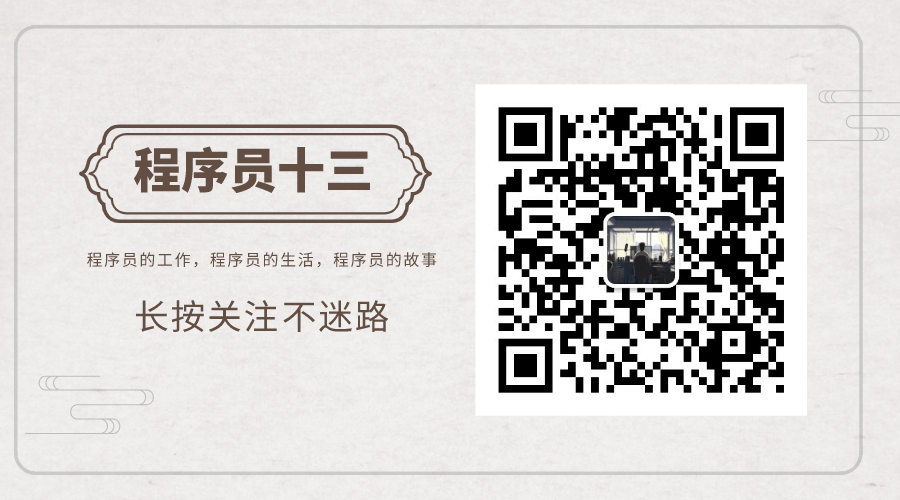
---
## 开发文档
### 《SpringBoot + Mybatis + Thymeleaf 搭建美观实用的个人博客》
[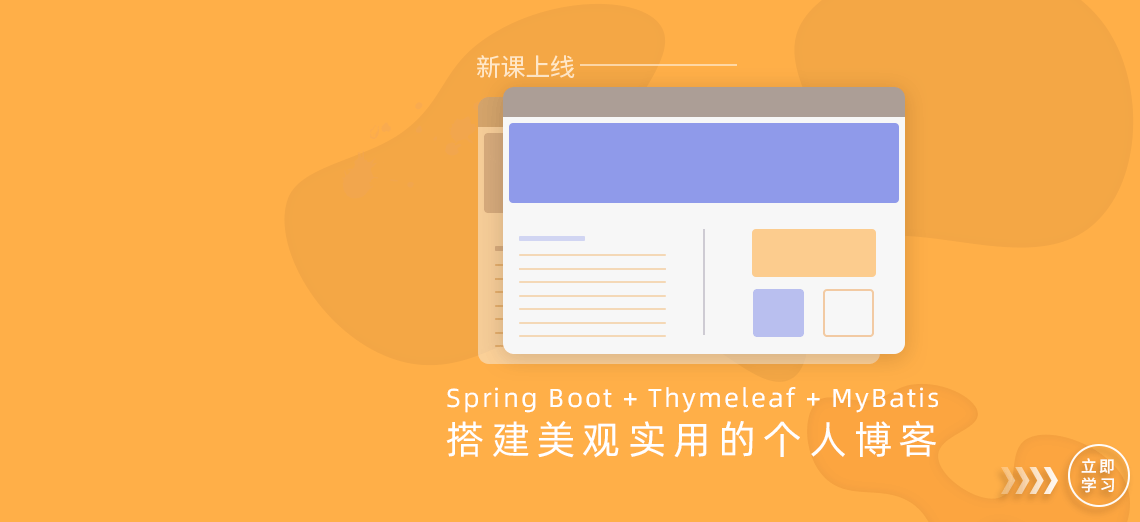](https://www.lanqiao.cn/courses/1367)
- [**第01课:Spring Boot 搭建简洁实用的个人博客系统导读**](https://www.lanqiao.cn/courses/1367)
- [第02课:快速构建 Spring Boot 应用](https://www.lanqiao.cn/courses/1367)
- [第03课:Spring Boot 项目开发之web项目开发讲解](https://www.lanqiao.cn/courses/1367)
- [第04课:Spring Boot 整合 Thymeleaf 模板引擎](https://www.lanqiao.cn/courses/1367)
- [第05课:Spring Boot 处理文件上传及路径回显](https://www.lanqiao.cn/courses)
- [第06课:Spring Boot 自动配置数据源及操作数据库](https://www.lanqiao.cn/courses/1367)
- [第07课:Spring Boot 整合 MyBatis 操作数据库](https://www.lanqiao.cn/courses/1367)
- [第08课:Mybatis-Generator 自动生成代码](https://www.lanqiao.cn/courses/1367)
- [第09课:Spring Boot 中的事务处理](https://www.lanqiao.cn/courses/1367)
- [第10课:Spring Boot 项目实践之 Ajax 技术使用教程](https://www.lanqiao.cn/courses/1367)
- [第11课:Spring Boot 项目实践之 RESTful API 设计与实现](https://www.lanqiao.cn/courses/1367)
- [第12课:Spring Boot 博客系统项目开发之分页功能实现](https://www.lanqiao.cn/courses/1367)
- [第13课:Spring Boot 博客系统项目开发之验证码功能](https://www.lanqiao.cn/courses/1367)
- [第14课:Spring Boot 博客系统项目开发之登录模块实现](https://www.lanqiao.cn/courses/1367)
- [第15课:Spring Boot 博客系统项目开发之登陆拦截器](https://www.lanqiao.cn/courses/1367)
- [第16课:Spring Boot 博客系统项目开发之分类功能实现](https://www.lanqiao.cn/courses/1367)
- [第17课:Spring Boot 博客系统项目开发之标签功能实现](https://www.lanqiao.cn/courses/1367)
- [第18课:Spring Boot 博客系统项目开发之文章编辑功能](https://www.lanqiao.cn/courses/1367)
- [第19课:Spring Boot 博客系统项目开发之文章编辑完善](https://www.lanqiao.cn/courses/1367)
- [第20课:Spring Boot 博客系统项目开发之文章模块实现](https://www.lanqiao.cn/courses/1367)
- [第21课:Spring Boot 博客系统项目开发之友链模块实现](https://www.lanqiao.cn/courses/1367)
- [第22课:Spring Boot 博客系统项目开发之网站首页制作](https://www.lanqiao.cn/courses/1367)
- [第23课:Spring Boot 博客系统项目开发之分页及侧边栏制作](https://www.lanqiao.cn/courses/1367)
- [第24课:Spring Boot 博客系统项目开发之搜索页面制作](https://www.lanqiao.cn/courses/1367)
- [第25课:Spring Boot 博客系统项目开发之文章详情页制作](https://www.lanqiao.cn/courses/1367)
- [第26课:Spring Boot 博客系统项目开发之错误页面制作](https://www.lanqiao.cn/courses/1367)
- [第27课:Spring Boot 博客系统项目开发之评论功能实现](https://www.lanqiao.cn/courses/1367)
- [第28课:Spring Boot 博客系统项目开发之项目打包部署](https://www.lanqiao.cn/courses/1367)
### 《SpringBoot + Mybatis + Thymeleaf 开发 BBS 论坛项目》
[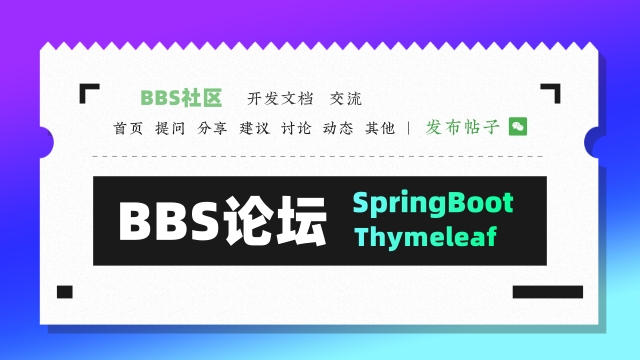](https://www.shiyanlou.com/courses/4830)
- [**第01课:Spring Boot 技术栈详解**](https://www.shiyanlou.com/courses/4830)
- [第02课:快速构建 Spring Boot 应用](https://www.shiyanlou.com/courses/4830)
- [第03课:Spring Boot 项目开发之Web项目开发讲解](https://www.shiyanlou.com/courses/4830)
- [第04课:Spring Boot 整合 Thymeleaf 模板引擎](https://www.shiyanlou.com/courses/4830)
- [第05课:Thymeleaf 语法详解](https://www.shiyanlou.com/courses/4830)
- [第06课:Spring Boot 自动配置数据源及操作数据库](https://www.shiyanlou.com/courses/4830)
- [第07课:Spring Boot 整合 MyBatis 操作数据库](https://www.shiyanlou.com/courses/4830)
- [第08课:分页功能的设计与实现](https://www.shiyanlou.com/courses/4830)
- [第09课:BBS论坛项目开发之验证码功能](https://www.shiyanlou.com/courses/4830)
- [第10课:BBS论坛项目开发之前端技术选型及源码目录详解](https://www.shiyanlou.com/courses/4830)
- [第11课:BBS论坛项目开发之用户注册模块实现](https://www.shiyanlou.com/courses/4830)
- [第12课:BBS论坛项目开发之用户登录模块实现](https://www.shiyanlou.com/courses/4830)
- [第13课:BBS论坛项目开发之用户登录拦截器](https://www.shiyanlou.com/courses/4830)
- [第14课:BBS论坛项目实战之处理文件上传及路径回显](https://www.shiyanlou.com/courses/4830)
- [第15课:BBS论坛项目实战之个人中心页面制作](https://www.shiyanlou.com/courses/4830)
- [第16课:富文本编辑器 wangEditor 整合](https://www.shiyanlou.com/courses/4830)
- [第17课:BBS论坛项目实战之论坛首页开发](https://www.shiyanlou.com/courses/4830)
- [第18课:BBS论坛项目实战之帖子发布功能开发](https://www.shiyanlou.com/courses/4830)
- [第19课:BBS论坛项目实战之帖子详情和修改功能开发](https://www.shiyanlou.com/courses/4830)
- [第20课:BBS论坛项目实战之评论功能开发](https://www.shiyanlou.com/courses/4830)
### 《Spring Boot 前后端分离项目实践》
[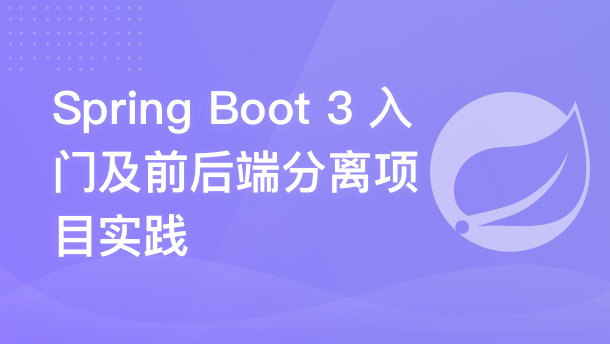](https://www.lanqiao.cn/courses/1244)
- [**开篇词:SpringBoot入门及前后端分离项目实践导读**](https://www.lanqiao.cn/courses/1244)
- [第02课:快速认识 Spring Boot 技术栈](https://www.lanqiao.cn/courses/1244)
- [第03课:开发环境搭建](https://www.lanqiao.cn/courses/1244)
- [第04课:快速构建 Spring Boot 应用](https://www.lanqiao.cn/courses/1244)
- [第05课:Spring Boot 之基础 web 功能开发](https://www.lanqiao.cn/courses)
- [第06课:Spring Boot 之数据库连接操作](https://www.lanqiao.cn/courses/1244)
- [第07课:Spring Boot 整合 MyBatis 操作数据库](https://www.lanqiao.cn/courses/1244)
- [第08课:Spring Boot 处理文件上传及路径回显](https://www.lanqiao.cn/courses/1244)
- [第09课:Spring Boot 项目实践之前后端分离详解](https://www.lanqiao.cn/courses/1244)
- [第10课:Spring Boot 项目实践之 Ajax 技术使用教程](https://www.lanqiao.cn/courses/1244)
- [第11课:Spring Boot 项目实践之 RESTful API 设计与实现](https://www.lanqiao.cn/courses/1244)
- [第12课:Spring Boot 项目实践之登录模块实现](https://www.lanqiao.cn/courses/1244)
- [第13课:Spring Boot 项目实践之分页功能实现](https://www.lanqiao.cn/courses/1244)
- [第14课:Spring Boot 项目实践之 jqgrid 分页整合](https://www.lanqiao.cn/courses/1244)
- [第15课:Spring Boot 项目实践之用户编辑功能实现](https://www.lanqiao.cn/courses/1244)
- [第16课:Spring Boot 项目实践之用户管理模块实现](https://www.lanqiao.cn/courses/1244)
- [第17课:Spring Boot 项目实践之图片管理模块](https://www.lanqiao.cn/courses/1244)
- [第18课:Spring Boot 项目实践之信息管理模块实现](https://www.lanqiao.cn/courses/1244)
### 《25 个实验带你轻松玩转 Spring Boot》
[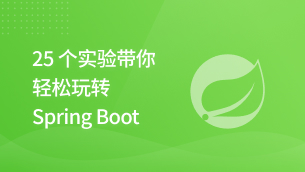](https://www.lanqiao.cn/courses/1274)
- [**开篇词:《25 个实验带你轻松玩转 Spring Boot》导读**](https://www.lanqiao.cn/courses/1274)
- [第02课:Spring Boot 项目开发环境搭建](https://www.lanqiao.cn/courses/1274)
- [第03课:快速构建 Spring Boot 应用](https://www.lanqiao.cn/courses/1274)
- [第04课:Spring Boot 基础功能开发](https://www.lanqiao.cn/courses/1274)
- [第05课:Spring Boot 项目开发之 web 项目开发讲解](https://www.lanqiao.cn/courses/1274)
- [第06课:Spring Boot 整合 JSP 开发 web 项目](https://www.lanqiao.cn/courses/1274)
- [第07课:模板引擎介绍及 Spring Boot 整合 Thymeleaf](https://www.lanqiao.cn/courses/1274)
- [第08课:Thymeleaf 语法详解](https://www.lanqiao.cn/courses/1274)
- [第09课:FreeMarker 模板引擎整合使用教程](https://www.lanqiao.cn/courses/1274)
- [第10课:Spring Boot 处理文件上传及路径回显](https://www.lanqiao.cn/courses/1274)
- [第11课:Spring Boot 自动配置数据源及操作数据库](https://www.lanqiao.cn/courses/1274)
- [第12课:Spring Boot 整合 Druid 数据源](https://www.lanqiao.cn/courses/1274)
- [第13课:Spring Boot 整合 MyBatis 操作数据库](https://www.lanqiao.cn/courses/1274)
- [第14课:Spring Boot 中的事务处理](https://www.lanqiao.cn/courses/1274)
- [第15课:Spring Boot 整合 Redis 操作缓存模块](https://www.lanqiao.cn/courses/1274)
- [第16课:Spring Boot 项目开发之实现定时任务](https://www.lanqiao.cn/courses/1274)
- [第17课:Spring Boot 自定义错误页面](https://www.lanqiao.cn/courses/1274)
- [第18课:Spring Boot 集成 Swagger 生成接口文档](https://www.lanqiao.cn/courses/1274)
- [第19课:Spring Boot 项目打包部署介绍](https://www.lanqiao.cn/courses/1274)
- [第20课:Spring Boot Admin 介绍及整合使用](https://www.lanqiao.cn/courses/1274)
- [第21课:Spring Boot 资讯管理信息系统开发实战(一)](https://www.lanqiao.cn/courses/1274)
- [第22课:Spring Boot 资讯管理信息系统开发实战(二)](https://www.lanqiao.cn/courses/1274)
- [第23课:Spring Boot 资讯管理信息系统开发实战(三)](https://www.lanqiao.cn/courses/1274)
- [第24课:Spring Boot 资讯管理信息系统开发实战(四)](https://www.lanqiao.cn/courses/1274)
---
## 交流
> 大家有任何问题或者建议都可以在 [issues](https://github.com/ZHENFENG13/spring-boot-projects/issues) 中反馈给我,我会慢慢完善这个 Spring Boot 仓库。
- 我的邮箱:2449207463@qq.com
- QQ技术交流群:719099151 784785001
| 0 |
google/closure-compiler | A JavaScript checker and optimizer. | closure-compiler javascript optimization typechecking | # [Google Closure Compiler](https://developers.google.com/closure/compiler/)
[](https://api.securityscorecards.dev/projects/github.com/google/closure-compiler)
[](https://github.com/google/closure-compiler/actions)
[](https://www.codetriage.com/google/closure-compiler)
[](https://github.com/google/closure-compiler/blob/master/code_of_conduct.md)
The [Closure Compiler](https://developers.google.com/closure/compiler/) is a
tool for making JavaScript download and run faster. It is a true compiler for
JavaScript. Instead of compiling from a source language to machine code, it
compiles from JavaScript to better JavaScript. It parses your JavaScript,
analyzes it, removes dead code and rewrites and minimizes what's left. It also
checks syntax, variable references, and types, and warns about common JavaScript
pitfalls.
## Important Caveats
1. Compilation modes other than `ADVANCED` were always an afterthought and we
have deprecated those modes. We believe that other tools perform comparably
for non-`ADVANCED` modes and are better integrated into the broader JS
ecosystem.
1. Closure Compiler is not suitable for arbitrary JavaScript. For `ADVANCED`
mode to generate working JavaScript, the input JS code must be written with
closure-compiler in mind.
1. Closure Compiler is a "whole world" optimizer. It expects to directly see or
at least receive information about every possible use of every global or
exported variable and every property name.
It will aggressively remove and rename variables and properties in order to
make the output code as small as possible. This will result in broken output
JS, if uses of global variables or properties are hidden from it.
Although one can write custom externs files to tell the compiler to leave
some names unchanged so they can safely be accessed by code that is not part
of the compilation, this is often tedious to maintain.
1. Closure Compiler property renaming requires you to consistently access a
property with either `obj[p]` or `obj.propName`, but not both.
When you access a property with square brackets (e.g. `obj[p]`) or using some
other indirect method like `let {p} = obj;` this hides the literal name of
the property being referenced from the compiler. It cannot know if
`obj.propName` is referring to the same property as `obj[p]`. In some cases
it will notice this problem and stop the compilation with an error. In other
cases it will rename `propName` to something shorter, without noticing this
problem, resulting in broken output JS code.
1. Closure Compiler aggressively inlines global variables and flattens chains
of property names on global variables (e.g. `myFoo.some.sub.property` ->
`myFoo$some$sub$property`), to make reasoning about them easier for detecting
unused code.
It tries to either back off from doing this or halt with an error when
doing it will generate broken JS output, but there are cases where it will
fail to recognize the problem and simply generate broken JS without warning.
This is much more likely to happen in code that was not explicitly written
with Closure Compiler in mind.
1. Closure compiler and the externs it uses by default assume that the target
environment is a web browser window.
WebWorkers are supported also, but the compiler will likely fail to warn
you if you try to use features that aren't actually available to a WebWorker.
Some externs files and features have been added to Closure Compiler to
support the NodeJS environment, but they are not actively supported and
never worked very well.
1. JavaScript that does not use the `goog.module()` and `goog.require()` from
`base.js` to declare and use modules is not well supported.
The ECMAScript `import` and `export` syntax did not exist until 2015.
Closure compiler and `closure-library` developed their own means for
declaring and using modules, and this remains the only well supported
way of defining modules.
The compiler does implement some understanding of ECMAScript modules,
but changing Google's projects to use the newer syntax has never offered
a benefit that was worth the cost of the change. Google's TypeScript code
uses ECMAScript modules, but they are converted to `goog.module()` syntax
before closure-compiler sees them. So, effectively the ECMAScript modules
support is unused within Google. This means we are unlikely to notice
or fix bugs in the support for ECMAScript modules.
Support for CommonJS modules as input was added in the past, but is not
used within Google, and is likely to be entirely removed sometime in 2024.
## Supported uses
Closure Compiler is used by Google projects to:
* Drastically reduce the code size of very large JavaScript applications
* Check the JS code for errors and for conformance to general and/or
project-specific best practices.
* Define user-visible messages in a way that makes it possible to replace
them with translated versions to create localized versions of an
application.
* Transpile newer JS features into a form that will run on browsers that
lack support for those features.
* Break the output application into chunks that may be individually loaded
as needed.
NOTE: These chunks are plain JavaScript scripts. They do not use the
ECMAScript `import` and `export` syntax.
To achieve these goals closure compiler places many restrictions on its input:
* Use `goog.module()` and `goog.require()` to declare and use modules.
Support for the `import` and `export` syntax added in ES6 is not actively
maintained.
* Use annotations in comments to declare type information and provide
information the compiler needs to avoid breaking some code patterns
(e.g. `@nocollapse` and `@noinline`).
* Either use only dot-access (e.g. `object.property`) or only use dynamic
access (e.g. `object[propertyName]` or `Object.keys(object)`) to access
the properties of a particular object type.
Mixing these will hide some uses of a property from the compiler, resulting
in broken output code when it renames the property.
* In general the compiler expects to see an entire application as a single
compilation. Interfaces must be carefully and explicitly constructed in
order to allow interoperation with code outside of the compilation unit.
The compiler assumes it can see all uses of all variables and properties
and will freely rename them or remove them if they appear unused.
* Use externs files to inform the compiler of any variables or properties
that it must not remove or rename.
There are default externs files declaring the standard JS and DOM global
APIs. More externs files are necessary if you are using less common
APIs or expect some external JavaScript code to access an API in the
code you are compiling.
## Getting Started
The easiest way to install the compiler is with [NPM](https://npmjs.com) or
[Yarn](https://yarnpkg.com):
```bash
yarn global add google-closure-compiler
# OR
npm i -g google-closure-compiler
```
The package manager will link the binary for you, and you can access the
compiler with:
```bash
google-closure-compiler
```
This starts the compiler in interactive mode. Type:
```javascript
var x = 17 + 25;
```
Hit `Enter`, then `Ctrl+Z` (on Windows) or `Ctrl+D` (on Mac/Linux), then `Enter`
again. The Compiler will respond with the compiled output (using `SIMPLE` mode
by default):
```javascript
var x=42;
```
#### Downloading from Maven Repository
A pre-compiled release of the compiler is also available via
[Maven](https://mvnrepository.com/artifact/com.google.javascript/closure-compiler).
### Basic usage
The Closure Compiler has many options for reading input from a file, writing
output to a file, checking your code, and running optimizations. Here is a
simple example of compressing a JS program:
```bash
google-closure-compiler --js file.js --js_output_file file.out.js
```
We get the **most benefit** from the compiler if we give it **all of our source
code** (see [Compiling Multiple Scripts](#compiling-multiple-scripts)), which
allows us to use `ADVANCED` optimizations:
```bash
google-closure-compiler -O ADVANCED rollup.js --js_output_file rollup.min.js
```
NOTE: The output below is just an example and not kept up-to-date. The
[Flags and Options wiki page](https://github.com/google/closure-compiler/wiki/Flags-and-Options)
is updated during each release.
To see all of the compiler's options, type:
```bash
google-closure-compiler --help
```
<table>
<thead>
<tr>
<th><code>--flag</code></th>
<th>Description</th>
</tr>
</thead>
<tbody>
<tr>
<td><code>--compilation_level (-O)</code></td>
<td>
Specifies the compilation level to use.
Options: <code>BUNDLE</code>, <code>WHITESPACE_ONLY</code>,
<code>SIMPLE</code> (default), <code>ADVANCED</code>
</td>
</tr>
<tr>
<td><code>--env</code></td>
<td>
Determines the set of builtin externs to load.
Options: <code>BROWSER</code>, <code>CUSTOM</code>.
Defaults to <code>BROWSER</code>.
</td>
</tr>
<tr>
<td><code>--externs</code></td>
<td>The file containing JavaScript externs. You may specify multiple</td>
</tr>
<tr>
<td><code>--js</code></td>
<td>
The JavaScript filename. You may specify multiple. The flag name is
optional, because args are interpreted as files by default. You may also
use minimatch-style glob patterns. For example, use
<code>--js='**.js' --js='!**_test.js'</code> to recursively include all
js files that do not end in <code>_test.js</code>
</td>
</tr>
<tr>
<td><code>--js_output_file</code></td>
<td>
Primary output filename. If not specified, output is written to stdout.
</td>
</tr>
<tr>
<td><code>--language_in</code></td>
<td>
Sets the language spec to which input sources should conform.
Options: <code>ECMASCRIPT3</code>, <code>ECMASCRIPT5</code>,
<code>ECMASCRIPT5_STRICT</code>, <code>ECMASCRIPT_2015</code>,
<code>ECMASCRIPT_2016</code>, <code>ECMASCRIPT_2017</code>,
<code>ECMASCRIPT_2018</code>, <code>ECMASCRIPT_2019</code>,
<code>STABLE</code>, <code>ECMASCRIPT_NEXT</code>
</td>
</tr>
<tr>
<td><code>--language_out</code></td>
<td>
Sets the language spec to which output should conform.
Options: <code>ECMASCRIPT3</code>, <code>ECMASCRIPT5</code>,
<code>ECMASCRIPT5_STRICT</code>, <code>ECMASCRIPT_2015</code>,
<code>ECMASCRIPT_2016</code>, <code>ECMASCRIPT_2017</code>,
<code>ECMASCRIPT_2018</code>, <code>ECMASCRIPT_2019</code>,
<code>STABLE</code>
</td>
</tr>
<tr>
<td><code>--warning_level (-W)</code></td>
<td>Specifies the warning level to use.
Options: <code>QUIET</code>, <code>DEFAULT</code>, <code>VERBOSE</code>
</td>
</tr>
</tbody>
</table>
#### See the [Google Developers Site](https://developers.google.com/closure/compiler/docs/gettingstarted_app) for documentation including instructions for running the compiler from the command line.
### NodeJS API
You can access the compiler in a JS program by importing
`google-closure-compiler`:
```javascript
import closureCompiler from 'google-closure-compiler';
const { compiler } = closureCompiler;
new compiler({
js: 'file-one.js',
compilation_level: 'ADVANCED'
});
```
This package will provide programmatic access to the native Graal binary in most
cases, and will fall back to the Java version otherwise.
#### Please see the [closure-compiler-npm](https://github.com/google/closure-compiler-npm/tree/master/packages/google-closure-compiler) repository for documentation on accessing the compiler in JS.
## Compiling Multiple Scripts
If you have multiple scripts, you should compile them all together with one
compile command.
```bash
google-closure-compiler in1.js in2.js in3.js --js_output_file out.js
```
You can also use minimatch-style globs.
```bash
# Recursively include all js files in subdirs
google-closure-compiler 'src/**.js' --js_output_file out.js
# Recursively include all js files in subdirs, excluding test files.
# Use single-quotes, so that bash doesn't try to expand the '!'
google-closure-compiler 'src/**.js' '!**_test.js' --js_output_file out.js
```
The Closure Compiler will concatenate the files in the order they're passed at
the command line.
If you're using globs or many files, you may start to run into problems with
managing dependencies between scripts. In this case, you should use the
included [lib/base.js](lib/base.js) that provides functions for enforcing
dependencies between scripts (namely `goog.module` and `goog.require`). Closure
Compiler will re-order the inputs automatically.
## Closure JavaScript Library
The Closure Compiler releases with [lib/base.js](lib/base.js) that provides
JavaScript functions and variables that serve as primitives enabling certain
features of the Closure Compiler. This file is a derivative of the
[identically named base.js](https://github.com/google/closure-library/blob/7818ff7dc0b53555a7fb3c3427e6761e88bde3a2/closure/goog/base.js)
in the
[soon-to-be deprecated](https://github.com/google/closure-library/issues/1214)
Closure Library. This `base.js` will be supported by Closure Compiler going
forward and may receive new features. It was designed to only retain its
perceived core parts.
## Getting Help
1. Post in the
[Closure Compiler Discuss Group](https://groups.google.com/forum/#!forum/closure-compiler-discuss).
2. Ask a question on
[Stack Overflow](https://stackoverflow.com/questions/tagged/google-closure-compiler).
3. Consult the [FAQ](https://github.com/google/closure-compiler/wiki/FAQ).
## Building the Compiler
To build the compiler yourself, you will need the following:
Prerequisite | Description
-------------------------------------------------------------------------- | -----------
[Java 11 or later](https://java.com) | Used to compile the compiler's source code.
[Git](https://git-scm.com/) | Used by Bazel to download dependencies.
[Bazelisk](https://bazel.build/install/bazelisk) | Used to build the various compiler targets.
### Installing Bazelisk
Bazelisk is a wrapper around Bazel that dynamically loads the appropriate
version of Bazel for a given repository. Using it prevents spurious errors that
result from using the wrong version of Bazel to build the compiler, as well as
makes it easy to use different Bazel versions for other projects.
Bazelisk is available through many package managers. Feel free to use whichever
you're most comfortable with.
[Instructions for installing Bazelisk](https://bazel.build/install/bazelisk).
### Building from a terminal
```bash
$ bazelisk build //:compiler_uberjar_deploy.jar
# OR to build everything
$ bazelisk build //:all
```
### Testing from a terminal
Tests can be executed in a similar way. The following command will run all tests
in the repo.
```bash
$ bazelisk test //:all
```
There are hundreds of individual test targets, so it will take a few
minutes to run all of them. While developing, it's usually better to specify
the exact tests you're interested in.
```bash
bazelisk test //:$path_to_test_file
```
### Building from an IDE
See [Bazel IDE Integrations](https://docs.bazel.build/versions/master/ide.html).
### Running
Once the compiler has been built, the compiled JAR will be in the `bazel-bin/`
directory. You can access it with a call to `java -jar ...` or by using the
package.json script:
```bash
# java -jar bazel-bin/compiler_uberjar_deploy.jar [...args]
yarn compile [...args]
```
#### Running using Eclipse
1. Open the class `src/com/google/javascript/jscomp/CommandLineRunner.java` or
create your own extended version of the class.
2. Run the class in Eclipse.
3. See the instructions above on how to use the interactive mode - but beware
of the
[bug](https://stackoverflow.com/questions/4711098/passing-end-of-transmission-ctrl-d-character-in-eclipse-cdt-console)
regarding passing "End of Transmission" in the Eclipse console.
## Contributing
### Contributor code of conduct
However you choose to contribute, please abide by our
[code of conduct](https://github.com/google/closure-compiler/blob/master/code_of_conduct.md) to
keep our community a healthy and welcoming place.
### Reporting a bug
1. First make sure that it is really a bug and not simply the way that Closure
Compiler works (especially true for ADVANCED_OPTIMIZATIONS).
* Check the
[official documentation](https://developers.google.com/closure/compiler/)
* Consult the [FAQ](https://github.com/google/closure-compiler/wiki/FAQ)
* Search on
[Stack Overflow](https://stackoverflow.com/questions/tagged/google-closure-compiler)
and in the
[Closure Compiler Discuss Group](https://groups.google.com/forum/#!forum/closure-compiler-discuss)
* Look through the list of
[compiler assumptions](https://github.com/google/closure-compiler/wiki/Compiler-Assumptions).
2. If you still think you have found a bug, make sure someone hasn't already
reported it. See the list of
[known issues](https://github.com/google/closure-compiler/issues).
3. If it hasn't been reported yet, post a new issue. Make sure to add enough
detail so that the bug can be recreated. The smaller the reproduction code,
the better.
### Suggesting a feature
1. Consult the [FAQ](https://github.com/google/closure-compiler/wiki/FAQ) to
make sure that the behaviour you would like isn't specifically excluded
(such as string inlining).
2. Make sure someone hasn't requested the same thing. See the list of
[known issues](https://github.com/google/closure-compiler/issues).
3. Read up on
[what type of feature requests are accepted](https://github.com/google/closure-compiler/wiki/FAQ#how-do-i-submit-a-feature-request-for-a-new-type-of-optimization).
4. Submit your request as an issue.
### Submitting patches
1. All contributors must sign a contributor license agreement (CLA). A CLA
basically says that you own the rights to any code you contribute, and that
you give us permission to use that code in Closure Compiler. You maintain
the copyright on that code. If you own all the rights to your code, you can
fill out an
[individual CLA](https://code.google.com/legal/individual-cla-v1.0.html). If
your employer has any rights to your code, then they also need to fill out a
[corporate CLA](https://code.google.com/legal/corporate-cla-v1.0.html). If
you don't know if your employer has any rights to your code, you should ask
before signing anything. By default, anyone with an @google.com email
address already has a CLA signed for them.
2. To make sure your changes are of the type that will be accepted, ask about
your patch on the
[Closure Compiler Discuss Group](https://groups.google.com/forum/#!forum/closure-compiler-discuss)
3. Fork the repository.
4. Make your changes. Check out our
[coding conventions](https://github.com/google/closure-compiler/wiki/Contributors#coding-conventions)
for details on making sure your code is in correct style.
5. Submit a pull request for your changes. A project developer will review your
work and then merge your request into the project.
## Closure Compiler License
Copyright 2009 The Closure Compiler Authors.
Licensed under the Apache License, Version 2.0 (the "License"); you may not use
this file except in compliance with the License. You may obtain a copy of the
License at http://www.apache.org/licenses/LICENSE-2.0.
Unless required by applicable law or agreed to in writing, software distributed
under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
CONDITIONS OF ANY KIND, either express or implied. See the License for the
specific language governing permissions and limitations under the License.
## Dependency Licenses
### Rhino
<table>
<tr>
<td>Code Path</td>
<td>
<code>src/com/google/javascript/rhino</code>, <code>test/com/google/javascript/rhino</code>
</td>
</tr>
<tr>
<td>URL</td>
<td>https://developer.mozilla.org/en-US/docs/Mozilla/Projects/Rhino</td>
</tr>
<tr>
<td>Version</td>
<td>1.5R3, with heavy modifications</td>
</tr>
<tr>
<td>License</td>
<td>Netscape Public License and MPL / GPL dual license</td>
</tr>
<tr>
<td>Description</td>
<td>A partial copy of Mozilla Rhino. Mozilla Rhino is an
implementation of JavaScript for the JVM. The JavaScript parse tree data
structures were extracted and modified significantly for use by Google's
JavaScript compiler.</td>
</tr>
<tr>
<td>Local Modifications</td>
<td>The packages have been renamespaced. All code not
relevant to the parse tree has been removed. A JsDoc parser and static typing
system have been added.</td>
</tr>
</table>
### Args4j
<table>
<tr>
<td>URL</td>
<td>http://args4j.kohsuke.org/</td>
</tr>
<tr>
<td>Version</td>
<td>2.33</td>
</tr>
<tr>
<td>License</td>
<td>MIT</td>
</tr>
<tr>
<td>Description</td>
<td>args4j is a small Java class library that makes it easy to parse command line
options/arguments in your CUI application.</td>
</tr>
<tr>
<td>Local Modifications</td>
<td>None</td>
</tr>
</table>
### Guava Libraries
<table>
<tr>
<td>URL</td>
<td>https://github.com/google/guava</td>
</tr>
<tr>
<td>Version</td>
<td>31.0.1</td>
</tr>
<tr>
<td>License</td>
<td>Apache License 2.0</td>
</tr>
<tr>
<td>Description</td>
<td>Google's core Java libraries.</td>
</tr>
<tr>
<td>Local Modifications</td>
<td>None</td>
</tr>
</table>
### JSR 305
<table>
<tr>
<td>URL</td>
<td>https://github.com/findbugsproject/findbugs</td>
</tr>
<tr>
<td>Version</td>
<td>3.0.1</td>
</tr>
<tr>
<td>License</td>
<td>BSD License</td>
</tr>
<tr>
<td>Description</td>
<td>Annotations for software defect detection.</td>
</tr>
<tr>
<td>Local Modifications</td>
<td>None</td>
</tr>
</table>
### JUnit
<table>
<tr>
<td>URL</td>
<td>http://junit.org/junit4/</td>
</tr>
<tr>
<td>Version</td>
<td>4.13</td>
</tr>
<tr>
<td>License</td>
<td>Common Public License 1.0</td>
</tr>
<tr>
<td>Description</td>
<td>A framework for writing and running automated tests in Java.</td>
</tr>
<tr>
<td>Local Modifications</td>
<td>None</td>
</tr>
</table>
### Protocol Buffers
<table>
<tr>
<td>URL</td>
<td>https://github.com/google/protobuf</td>
</tr>
<tr>
<td>Version</td>
<td>3.0.2</td>
</tr>
<tr>
<td>License</td>
<td>New BSD License</td>
</tr>
<tr>
<td>Description</td>
<td>Supporting libraries for protocol buffers,
an encoding of structured data.</td>
</tr>
<tr>
<td>Local Modifications</td>
<td>None</td>
</tr>
</table>
### RE2/J
<table>
<tr>
<td>URL</td>
<td>https://github.com/google/re2j</td>
</tr>
<tr>
<td>Version</td>
<td>1.3</td>
</tr>
<tr>
<td>License</td>
<td>New BSD License</td>
</tr>
<tr>
<td>Description</td>
<td>Linear time regular expression matching in Java.</td>
</tr>
<tr>
<td>Local Modifications</td>
<td>None</td>
</tr>
</table>
### Truth
<table>
<tr>
<td>URL</td>
<td>https://github.com/google/truth</td>
</tr>
<tr>
<td>Version</td>
<td>1.1</td>
</tr>
<tr>
<td>License</td>
<td>Apache License 2.0</td>
</tr>
<tr>
<td>Description</td>
<td>Assertion/Proposition framework for Java unit tests</td>
</tr>
<tr>
<td>Local Modifications</td>
<td>None</td>
</tr>
</table>
### Ant
<table>
<tr>
<td>URL</td>
<td>https://ant.apache.org/bindownload.cgi</td>
</tr>
<tr>
<td>Version</td>
<td>1.10.11</td>
</tr>
<tr>
<td>License</td>
<td>Apache License 2.0</td>
</tr>
<tr>
<td>Description</td>
<td>Ant is a Java based build tool. In theory it is kind of like "make"
without make's wrinkles and with the full portability of pure java code.</td>
</tr>
<tr>
<td>Local Modifications</td>
<td>None</td>
</tr>
</table>
### GSON
<table>
<tr>
<td>URL</td>
<td>https://github.com/google/gson</td>
</tr>
<tr>
<td>Version</td>
<td>2.9.1</td>
</tr>
<tr>
<td>License</td>
<td>Apache license 2.0</td>
</tr>
<tr>
<td>Description</td>
<td>A Java library to convert JSON to Java objects and vice-versa</td>
</tr>
<tr>
<td>Local Modifications</td>
<td>None</td>
</tr>
</table>
### Node.js Closure Compiler Externs
<table>
<tr>
<td>Code Path</td>
<td><code>contrib/nodejs</code></td>
</tr>
<tr>
<td>URL</td>
<td>https://github.com/dcodeIO/node.js-closure-compiler-externs</td>
</tr>
<tr>
<td>Version</td>
<td>e891b4fbcf5f466cc4307b0fa842a7d8163a073a</td>
</tr>
<tr>
<td>License</td>
<td>Apache 2.0 license</td>
</tr>
<tr>
<td>Description</td>
<td>Type contracts for NodeJS APIs</td>
</tr>
<tr>
<td>Local Modifications</td>
<td>Substantial changes to make them compatible with NpmCommandLineRunner.</td>
</tr>
</table>
| 0 |
alfio-event/alf.io | alf.io - The open source ticket reservation system for conferences, trade shows, workshops, meetups | alfio conferences event java meetups reservation ticket ticketing workshops | [alf.io](https://alf.io)
========
The open source ticket reservation system.
[Alf.io](https://alf.io) <small>([[ˈalfjo]](https://en.m.wikipedia.org/wiki/Alfio))</small> is a free and open source event attendance management system, developed for event organizers who care about privacy, security and fair pricing policy for their customers.
[](https://github.com/alfio-event/alf.io/actions)
[](https://sonarcloud.io/summary/overall?id=alfio-event_alf.io)
[](https://sonarcloud.io/summary/overall?id=alfio-event_alf.io)
[](https://sonarcloud.io/summary/overall?id=alfio-event_alf.io)
[](https://opencollective.com/alfio)
[](https://hub.docker.com/r/alfio/alf.io/tags)
[](https://www.codetriage.com/alfio-event/alf.io)
## Prerequisites
You should have installed Java version **17** (e.g. [Oracle's](http://www.oracle.com/technetwork/java/javase/downloads/index.html), [OpenJDK](http://openjdk.java.net/install/), or any other distribution) to build and run alf.io. Please note that for the build process the JDK is required.
Postgresql version 10 or later.
Additionally, the database user that creates and uses the tables should not be a "SUPERUSER", or else the row security policy checks will not be applied.
> [!NOTE]
> As the work for Alf.io [v2](https://github.com/alfio-event/alf.io/milestones) has started, this branch may contain **unstable** and **untested** code.
> If you want to build and deploy alf.io by yourself, please start from a [Released version](https://github.com/alfio-event/alf.io/releases).
## Run on your machine
### Gradle Build
This build includes a copy of the Gradle wrapper. You don't have to have Gradle installed on your system to build
the project. Simply execute the wrapper along with the appropriate task, for example
```
./gradlew clean
```
#### Running with multiple profiles
You must specify a project property at the command line, such as
```
./gradlew -Pprofile=dev :bootRun
```
The local "bootRun" task has the following prerequisites:
- a PostgreSQL (version 10 or later) instance up and running on localhost:5432
- a _postgres_ user having a password: _password_
- a database named _alfio_
once started, alf.io will create all the required tables in the database, and be available at http://localhost:8080/admin. You can log in using the default Username _admin_ and the password which was printed on the console.
The following profiles are supported
* `dev`
* `dev-pgsql`
* `docker-test`
You can get a list of all supported Gradle tasks by running
```
./gradlew tasks --all
```
You can configure additional System properties (if you need them) by creating the following file and putting into it one property per line:
```
vi custom.jvmargs
```
Please be aware that since this file could contain sensitive information (such as Google Maps private API key) it will be automatically ignored by git.
#### For debug
Add a new line with: `-agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=5005` in custom.jvmargs
## Developing alf.io
Importing the Gradle project into Intellij and Eclipse both work.
**Notes**:
- this project uses [Project Lombok](https://projectlombok.org/). You will need to install the corresponding Lombok plugin for integration into your IDE.
- this project uses [TestContainers](https://testcontainers.org) to run integration tests against a real PostgreSQL database. Please make sure that your configuration meets [their requirements](https://www.testcontainers.org/supported_docker_environment/)
<details><summary>How to configure TestContainers with Podman (Fedora 34)</summary>
<p>
As TestContainers expect the docker socket for managing the containers, you will need to do the following <a href="https://github.com/containers/podman/issues/7927#issuecomment-732676422" target="_blank" rel="noopener">(see original issue for details)</a>:
Define the 2 env. variable:
```
export TESTCONTAINERS_RYUK_DISABLED=true
export DOCKER_HOST=unix:///run/user/1000/podman/podman.sock
```
And run in another console:
```
podman system service -t 0
```
To be noted:
- for unknown reason, the first time podman download the missing images, testcontainers will fail. Run another time and it will work.
- in theory, with systemd+socket activation the service should start automatically, but currently I was not able to make it works.
</p>
</details>
## Check dependencies to update
`./gradlew dependencyUpdates`
## Docker
Container images are available on https://hub.docker.com/r/alfio/alf.io/tags.
alf.io can also be run with Docker Compose (*experimental*):
docker-compose up
Running alf.io in production using Docker compose is not officially supported.
However, if you decide to do so, then you need to make a couple of changes:
* Add a mapping for port `8443`
* Handle SSL termination (e.g. with something like `tutum/haproxy`)
* Remove the `SPRING_PROFILES_ACTIVE: dev` environment variable
### Test alf.io application
* Check alfio logs: `docker logs alfio`
* Copy admin password in a secure place
* Get IP of your docker container: (only on Mac/Windows, on Linux the proxy will bind directly on your public IP)
* `boot2docker IP` on Mac/Windows
* Open browser at: `https://DOCKER_IP/admin`
* Insert user admin and the password you just copied
### Generate a new version of the alfio/alf.io docker image
#### Build application and Dockerfile
```
./gradlew distribution
```
Alternatively, you can use Docker (*experimental*):
```
docker run --rm -u gradle -v "$PWD":/home/gradle/project -w /home/gradle/project gradle:7.0.0-jdk11 gradle --no-daemon distribution -x test
```
Please note that at the moment the command above performs the build without running the automated tests.
Use it at your own risk.
#### Create docker image:
```
docker build -t alfio/alf.io ./build/dockerize
```
### About the included AppleWWDRCAG4.cer
The certificate at src/main/resources/alfio/certificates/AppleWWDRCAG4.cer has been imported for https://github.com/ryantenney/passkit4j#usage functionality.
It will expire the 2030-10-12 (YYYY-MM-DD - as of https://www.apple.com/certificateauthority/).
## Available spring profiles:
- dev: enable dev mode
- spring-boot: added when launched by spring-boot
- demo: enable demo mode, the accounts for the admin will be created on the fly
- disable-jobs: disable jobs
## Contributors
### Code Contributors
This project exists thanks to all the people who contribute.
<a href="https://github.com/alfio-event/alf.io/graphs/contributors"><img src="https://opencollective.com/alfio/contributors.svg?width=890&button=false" /></a>
### Translation Contributors (POEditor)
A big "Thank you" goes also to our translators, who help us on [POEditor](https://github.com/alfio-event/alf.io/tree/master/src/main/resources/alfio/i18n):
(we show the complete name/profile only if we have received explicit consent to do so)
| Language | Name | Github | Twitter |
| ------------- |:--------------| -----: |---|
| Dutch (nl) | Matthjis | | |
| Turkish (tr) | Dilek | | |
| Spanish (es) | Mario Varona | [@mvarona](https://www.github.com/mvarona) | [@MarioVarona](https://www.twitter.com/MarioVarona) |
| Spanish (es) | Sergi Almar | [@salmar](https://www.github.com/salmar) | [@sergialmar](https://www.twitter.com/sergialmar) |
| Spanish (es) | Jeremias | | |
| Bulgarian (bg) | Martin Zhekov | [@Martin03](https://www.github.com/Martin03) | [@MartensZone](https://www.twitter.com/MartensZone) |
| Portuguese (pt) | Hugo | | |
| Swedish (sv) | Johan | | |
| Romanian (ro) | Daniel | | |
| Polish (pl) | Pawel | | |
| Danish (da) | Sune | | |
translations completed but not yet integrated (WIP)
| Language | Name | Github | Twitter |
| ------------- |:--------------| -----: |---|
| Japanese (jp) | Martin | | |
| Chinese (Taiwan) (cn_TW) | Yu-cheng, Lin | | |
### Sponsors
This project is sponsored by:
<a href="https://swicket.io" target="_blank">
<img alt="Swicket" src="https://swicket.io/logo-web.png" width="200">
</a><br/>
<a href="https://www.browserstack.com/open-source" target="_blank"><img alt="Powered by BrowserStack" src="https://user-images.githubusercontent.com/2320747/150974875-769c7085-f8bf-49b8-aff9-b650231300eb.jpg" width="200"/> Open Source program</a>
<br><br>
<img alt="Exteso" src="https://alf.io/img/logos/exteso_logo.jpg" width="150">
<a href="https://www.starplane.it/" target="_blank">
<img alt="Starplane" src="https://alf.io/img/logos/starplane.png" width="120">
</a>
<a href="https://www.amicidelticino.ch/" target="_blank">
<img alt="Amici del Ticino" src="https://alf.io/img/logos/amicidelticino-logo.png" width="120">
</a>
<a href="https://www.netlify.com/" target="_blank">
<img alt="Netlify" src="https://www.netlify.com/img/global/badges/netlify-color-accent.svg" width="120">
</a>
### Financial Contributors
Become a financial contributor and help us sustain our community. [[Contribute](https://opencollective.com/alfio/contribute)]
#### Individuals
<a href="https://opencollective.com/alfio"><img src="https://opencollective.com/alfio/individuals.svg?width=890"></a>
#### Organizations
Support this project with your organization. Your logo will show up here with a link to your website. [[Contribute](https://opencollective.com/alfio/contribute)]
<a href="https://opencollective.com/alfio/organization/0/website"><img src="https://images.opencollective.com/spring_io/01a0fe1/logo.png" height="100"></a>
<a href="https://opencollective.com/alfio/organization/1/website"><img src="https://images.opencollective.com/salesforce/ca8f997/logo/256.png" height="100"></a>
<a href="https://opencollective.com/alfio/organization/2/website"><img src="https://opencollective.com/alfio/organization/2/avatar.svg"></a>
| 0 |
apache/lucene | Apache Lucene open-source search software | backend information-retrieval java lucene nosql search search-engine | <!--
Licensed to the Apache Software Foundation (ASF) under one or more
contributor license agreements. See the NOTICE file distributed with
this work for additional information regarding copyright ownership.
The ASF licenses this file to You under the Apache License, Version 2.0
the "License"); you may not use this file except in compliance with
the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-->
# Apache Lucene
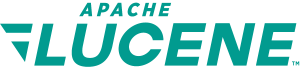
Apache Lucene is a high-performance, full-featured text search engine library
written in Java.
[](https://ci-builds.apache.org/job/Lucene/job/Lucene-Artifacts-main/)
## Online Documentation
This README file only contains basic setup instructions. For more
comprehensive documentation, visit:
- Latest Releases: <https://lucene.apache.org/core/documentation.html>
- Nightly: <https://ci-builds.apache.org/job/Lucene/job/Lucene-Artifacts-main/javadoc/>
- Build System Documentation: [help/](./help/)
- Developer Documentation: [dev-docs/](./dev-docs/)
- Migration Guide: [lucene/MIGRATE.md](./lucene/MIGRATE.md)
## Building
### Basic steps:
1. Install OpenJDK 21.
2. Clone Lucene's git repository (or download the source distribution).
3. Run gradle launcher script (`gradlew`).
We'll assume that you know how to get and set up the JDK - if you don't, then we suggest starting at https://jdk.java.net/ and learning more about Java, before returning to this README.
See [Contributing Guide](./CONTRIBUTING.md) for details.
## Contributing
Bug fixes, improvements and new features are always welcome!
Please review the [Contributing to Lucene
Guide](./CONTRIBUTING.md) for information on
contributing.
## Discussion and Support
- [Users Mailing List](https://lucene.apache.org/core/discussion.html#java-user-list-java-userluceneapacheorg)
- [Developers Mailing List](https://lucene.apache.org/core/discussion.html#developer-lists)
- IRC: `#lucene` and `#lucene-dev` on freenode.net
| 0 |
openjdk/jdk | JDK main-line development https://openjdk.org/projects/jdk | java jvm openjdk | # Welcome to the JDK!
For build instructions please see the
[online documentation](https://openjdk.org/groups/build/doc/building.html),
or either of these files:
- [doc/building.html](doc/building.html) (html version)
- [doc/building.md](doc/building.md) (markdown version)
See <https://openjdk.org/> for more information about the OpenJDK
Community and the JDK and see <https://bugs.openjdk.org> for JDK issue
tracking.
| 0 |
android/testing-samples | A collection of samples demonstrating different frameworks and techniques for automated testing | null | Android testing samples
===================================
A collection of samples demonstrating different frameworks and techniques for automated testing.
### Espresso Samples
**[BasicSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/BasicSample)** - Basic Espresso sample
**[CustomMatcherSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/CustomMatcherSample)** - Shows how to extend Espresso to match the *hint* property of an EditText
**[DataAdapterSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/DataAdapterSample)** - Showcases the `onData()` entry point for Espresso, for lists and AdapterViews
**[FragmentScenarioSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/FragmentScenarioSample)** - Basic usage of `FragmentScenario` with Espresso.
**[IdlingResourceSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/IdlingResourceSample)** - Synchronization with background jobs
**[IntentsBasicSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/IntentsBasicSample)** - Basic usage of `intended()` and `intending()`
**[IntentsAdvancedSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/IntentsAdvancedSample)** - Simulates a user fetching a bitmap using the camera
**[MultiWindowSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/MultiWindowSample)** - Shows how to point Espresso to different windows
**[RecyclerViewSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/RecyclerViewSample)** - RecyclerView actions for Espresso
**[ScreenshotSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/ScreenshotSample)** - Screenshot capturing and saving using Espresso and androidx.test.core APIs
**[WebBasicSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/WebBasicSample)** - Use Espresso-web to interact with WebViews
**[BasicSampleBundled](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/BasicSampleBundled)** - Basic sample for Eclipse and other IDEs
**[MultiProcessSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/MultiProcessSample)** - Showcases how to use multiprocess Espresso.
### UiAutomator Sample
**[BasicSample](https://github.com/googlesamples/android-testing/tree/main/ui/uiautomator/BasicSample)** - Basic UI Automator sample
### AndroidJUnitRunner Sample
**[AndroidJunitRunnerSample](https://github.com/googlesamples/android-testing/tree/main/runner/AndroidJunitRunnerSample)** - Showcases test annotations, parameterized tests and testsuite creation
### JUnit4 Rules Sample
**All previous samples use ActivityTestRule or IntentsTestRule but there's one specific to ServiceTestRule:
**[BasicSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/BasicSample)** - Simple usage of `ActivityTestRule`
**[IntentsBasicSample](https://github.com/googlesamples/android-testing/blob/main/ui/espresso/IntentsBasicSample)** - Simple usage of `IntentsTestRule`
**[ServiceTestRuleSample](https://github.com/googlesamples/android-testing/tree/main/integration/ServiceTestRuleSample)** - Simple usage of `ServiceTestRule`
Prerequisites
--------------
- Android SDK v28
- Android Build Tools v28.03
Getting Started
---------------
These samples use the Gradle build system. To build a project, enter the project directory and use the `./gradlew assemble` command or use "Import Project" in Android Studio.
- Use `./gradlew connectedAndroidTest` to run the tests on a connected emulator or device.
- Use `./gradlew test` to run the unit test on your local host.
There is a top-level `build.gradle` file if you want to build and test all samples from the root directory. This is mostly helpful to build on a CI (Continuous Integration) server.
AndroidX Test Library
---------------
Many of these samples use the AndroidX Test Library. Visit the [Testing site on developer.android.com](https://developer.android.com/training/testing) for more information.
Experimental Bazel Support
--------------------------
[](https://buildkite.com/bazel/android-testing)
Some of these samples can be tested with [Bazel](https://bazel.build) on Linux. These samples contain a `BUILD.bazel` file, which is similar to a `build.gradle` file. The external dependencies are defined in the top level `WORKSPACE` file.
This is __experimental__ feature. To run the tests, please install the latest version of Bazel (0.12.0 or later) by following the [instructions on the Bazel website](https://docs.bazel.build/versions/master/install-ubuntu.html).
### Bazel commands
```
# Clone the repository if you haven't.
$ git clone https://github.com/google/android-testing
$ cd android-testing
# Edit the path to your local SDK at the top of the WORKSPACE file
$ $EDITOR WORKSPACE
# Test everything in a headless mode (no graphical display)
$ bazel test //... --config=headless
# Test a single test, e.g. ui/espresso/BasicSample/BUILD.bazel
$ bazel test //ui/uiautomator/BasicSample:BasicSampleInstrumentationTest_21_x86 --config=headless
# Query for all android_instrumentation_test targets
$ bazel query 'kind(android_instrumentation_test, //...)'
//ui/uiautomator/BasicSample:BasicSampleInstrumentationTest_23_x86
//ui/uiautomator/BasicSample:BasicSampleInstrumentationTest_22_x86
//ui/uiautomator/BasicSample:BasicSampleInstrumentationTest_21_x86
//ui/uiautomator/BasicSample:BasicSampleInstrumentationTest_19_x86
//ui/espresso/RecyclerViewSample:RecyclerViewSampleInstrumentationTest_23_x86
//ui/espresso/RecyclerViewSample:RecyclerViewSampleInstrumentationTest_22_x86
//ui/espresso/RecyclerViewSample:RecyclerViewSampleInstrumentationTest_21_x86
//ui/espresso/RecyclerViewSample:RecyclerViewSampleInstrumentationTest_19_x86
//ui/espresso/MultiWindowSample:MultiWindowSampleInstrumentationTest_23_x86
//ui/espresso/MultiWindowSample:MultiWindowSampleInstrumentationTest_22_x86
...
# Test everything with GUI enabled
$ bazel test //... --config=gui
# Test with a local device or emulator. Ensure that `adb devices` lists the device.
$ bazel test //... --config=local_device
# If multiple devices are connected, add --device_serial_number=$identifier where $identifier is the name of the device in `adb devices`
$ bazel test //... --config=local_device --test_arg=--device_serial_number=$identifier
```
For more information, check out the documentation for [Android Instrumentation Tests in Bazel](https://docs.bazel.build/versions/master/android-instrumentation-test.html). You may also want to check out [Building an Android App with Bazel](https://docs.bazel.build/versions/master/tutorial/android-app.html), and the list of [Android Rules](https://docs.bazel.build/versions/master/be/android.html) in the Bazel Build Encyclopedia.
Known issues:
* Building of APKs is supported on Linux, Mac and Windows, but testing is only supported on Linux.
* `android_instrumentation_test.target_device` attribute still needs to be specified even if `--config=local_device` is used.
* If using a local device or emulator, the APKs are not uninstalled automatically after the test. Use this command to
remove the packages:
* `adb shell pm list packages com.example.android.testing | cut -d ':' -f 2 | tr -d '\r' | xargs -L1 -t adb uninstall`
Please file Bazel related issues against the [Bazel](https://github.com/bazelbuild/bazel) repository instead of this repository.
Support
-------
- Google+ Community: https://plus.google.com/communities/105153134372062985968
- Stack Overflow: http://stackoverflow.com/questions/tagged/android-testing
If you've found an error in this sample, please file an issue:
https://github.com/googlesamples/android-testing
Patches are encouraged, and may be submitted by forking this project and
submitting a pull request through GitHub. Please see CONTRIBUTING.md for more details.
License
-------
Copyright 2015 The Android Open Source Project, Inc.
Licensed to the Apache Software Foundation (ASF) under one or more contributor
license agreements. See the NOTICE file distributed with this work for
additional information regarding copyright ownership. The ASF licenses this
file to you under the Apache License, Version 2.0 (the "License"); you may not
use this file except in compliance with the License. You may obtain a copy of
the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
License for the specific language governing permissions and limitations under
the License.
| 0 |
kevin-wayne/algs4 | Algorithms, 4th edition textbook code and libraries | null | ## Overview
<IMG SRC="http://algs4.cs.princeton.edu/cover.png" align=right hspace=25 width=100 alt = "Algorithms 4/e textbook">
This <a href = "https://github.com/kevin-wayne/algs4">public repository</a>
contains the Java <a href = "http://algs4.cs.princeton.edu/code/">source code</a>
for the algorithms and clients in the textbook
<a href = "http://amzn.to/13VNJi7">Algorithms, 4th Edition</a> by Robert Sedgewick and Kevin Wayne.
The official version at <a href = "https://github.com/kevin-wayne/algs4">https://github.com/kevin-wayne/algs4</a>
is actively maintained and updated by the authors.
The programs are organized in the package <code>edu.princeton.cs.algs4</code>.
If you need only the class files (and not the source code), you can use
<a href = "http://algs4.cs.princeton.edu/code/algs4.jar">algs4.jar</a> instead.
<br>
## Design goals
Our original goal was to cover the <em>50 algorithms that every programmer should know</em>.
We use the word <em>programmer</em> to refer to anyone engaged in trying to accomplish
something with the help of a computer, including scientists, engineers, and applications
developers, not to mention college students in science, engineering, and computer science.
The code is optimized for clarity, portability, and efficiency. While some of our
implementations are as fast as (or faster than) their counterparts in <tt>java.util</tt>,
our main goal is to express the core algorithmic ideas in an elegant and simple manner.
While we embrace some advanced Java features (such as generics and iterators),
we avoid those that interfere with the exposition (such as inheritance and concurrency).
## Build managers
This repository is intended for use with either the <a href = "https://maven.apache.org">Maven</a>
or <a href = "https://gradle.org">Gradle</a> build managers.
It can be run from either the command line or integrated into
Eclipse, NetBeans, and IntelliJ.
You can also access it via <a href = "https://bintray.com/algs4/maven/algs4">Bintray</a>.
## Coursera Algorithms, Part I and II students
Feel free to use this public repository to develop solutions to the programming assignments.
However, please do not store solutions to programming assignments in public repositories.
## Copyright
Copyright © 2000–2023 by Robert Sedgewick and Kevin Wayne.
## License
This code is released under GPLv3.
## Contribute to this repository
This <a href = "http://algs4.cs.princeton.edu/code/wishlist.txt">wishlist.txt</a>
contains a list of algorithms and data structures that we would
like to add to the repository. Indeed, several of the algorithms and
data structures in this repository were contributed by others. If interested, please
follow the same style as the code in the repository and thoroughly test your
code before contacting us.
## Support for other programming languages
Some of the code in this repository has been translated to other languages:
<ul>
<li><a href = "https://github.com/garyaiki/Scala-Algorithms">Scala</a> by Gary Struthers
<li><a href = "https://github.com/nguyenqthai/Algs4Net">.NET</a> by Quoc Thai
<li><a href = "https://github.com/itu-algorithms/itu.algs4">Python</a> by ITU Copenhagen
<li><a href = "https://github.com/shellfly/algs4-py">Python</a> by Li Hao
<li><a href = "https://github.com/shellfly/algo">Go</a> by Li Hao
</ul>
## Credits
Thanks to Peter Korgan for Maven and Gradle support.
| 0 |
janishar/mit-deep-learning-book-pdf | MIT Deep Learning Book in PDF format (complete and parts) by Ian Goodfellow, Yoshua Bengio and Aaron Courville | book chapter clear deep-learning deeplearning excercises good learning lecture-notes linear-algebra machine machine-learning mit neural-network neural-networks pdf print printable thinking | [](https://github.com/janishar/mit-deep-learning-book-pdf/raw/master/complete-book-pdf/deeplearningbook.pdf)
[](https://github.com/janishar/mit-deep-learning-book-pdf/blob/master/complete-book-pdf/Ian%20Goodfellow%2C%20Yoshua%20Bengio%2C%20Aaron%20Courville%20-%20Deep%20Learning%20(2017%2C%20MIT).pdf)
# MIT Deep Learning Book (beautiful and flawless PDF version)
MIT Deep Learning Book in PDF format (complete and parts) by Ian Goodfellow, Yoshua Bengio and Aaron Courville.
## Project Starter Template
A good project structure is very important for data-science and data-analytics work. I have open-sourced a very effective repo with project starter template: [Repo Link](https://github.com/janishar/data-analytics-project-template)
[https://github.com/janishar/data-analytics-project-template](https://github.com/janishar/data-analytics-project-template)
# About The Author
You can connect with me here:
* [Janishar Ali](https://janisharali.com)
* [Twitter](https://twitter.com/janisharali)
* [YouTube Channel](https://www.youtube.com/@unusualcode)
## If this repository helps you in anyway, show your love :heart: by putting a :star: on this project :v:
### Deep Learning
An MIT Press book
Ian Goodfellow and Yoshua Bengio and Aaron Courville
This is the most comprehensive book available on the deep learning and available as free html book for reading at http://www.deeplearningbook.org/
**Comment on this book by Elon Musk**
>Written by three experts in the field, Deep Learning is the only comprehensive book on the subject." -- Elon Musk, cochair of OpenAI; cofounder and CEO of Tesla and SpaceX
**This is not available as PDF download. So, I have taken the prints of the HTML content and binded into a flawless PDF version of the book, as suggested by the website itself**
**http://www.deeplearningbook.org/ says:**
>What is the best way to print the HTML format?
>Printing seems to work best printing directly from the browser, using Chrome. Other browsers do not work as well.
**This repository contains**
1. The pdf version of the book which is available in html at http://www.deeplearningbook.org/
2. The book is available in chapter wise PDFs as well as complete book in PDF.
**Some useful links for this learning:**
1. [Exercises](http://www.deeplearningbook.org/exercises.html)
2. [Lecture Slides](http://www.deeplearningbook.org/lecture_slides.html)
3. [External links](http://www.deeplearningbook.org/external.html)
*If you like this book then buy a copy of it and keep it with you forever. This will help you and also support the authors and the people involved in the effort of bringing this beautiful piece of work to public. Buy it from amazon, It is not expensive ($72). [Amazon](https://www.amazon.com/Deep-Learning-Adaptive-Computation-Machine/dp/0262035618)*
```
An MIT Press book
Ian Goodfellow, Yoshua Bengio and Aaron Courville
The Deep Learning textbook is a resource intended to help students and practitioners
enter the field of machine learning in general and deep learning in particular.
The online version of the book is now complete and will remain available online for free.
Citing the book
To cite this book, please use this bibtex entry:
@book{Goodfellow-et-al-2016,
title={Deep Learning},
author={Ian Goodfellow and Yoshua Bengio and Aaron Courville},
publisher={MIT Press},
note={\url{http://www.deeplearningbook.org}},
year={2016}
}
```
| 0 |
influxdata/influxdb-java | Java client for InfluxDB | null | # influxdb-java
[](https://github.com/influxdata/influxdb-java/actions)
[](http://codecov.io/github/influxdata/influxdb-java?branch=master)
[](https://codeclimate.com/github/influxdata/influxdb-java)
This is the official (and community-maintained) Java client library for [InfluxDB](https://www.influxdata.com/products/influxdb-overview/) (1.x), the open source time series database that is part of the TICK (Telegraf, InfluxDB, Chronograf, Kapacitor) stack.
For InfluxDB 3.0 users, this library is succeeded by the lightweight [v3 client library](https://github.com/InfluxCommunity/influxdb3-java).
_Note: This library is for use with InfluxDB 1.x and [2.x compatibility API](https://docs.influxdata.com/influxdb/v2.0/reference/api/influxdb-1x/). For full supports of InfluxDB 2.x features, please use the [influxdb-client-java](https://github.com/influxdata/influxdb-client-java) client._
## Adding the library to your project
The library artifact is published in Maven central, available at [https://search.maven.org/artifact/org.influxdb/influxdb-java](https://search.maven.org/artifact/org.influxdb/influxdb-java).
### Release versions
Maven dependency:
```xml
<dependency>
<groupId>org.influxdb</groupId>
<artifactId>influxdb-java</artifactId>
<version>${influxdbClient.version}</version>
</dependency>
```
Gradle dependency:
```bash
compile group: 'org.influxdb', name: 'influxdb-java', version: "${influxdbClientVersion}"
```
## Features
* Querying data using:
* [Influx Query Language (InfluxQL)](https://docs.influxdata.com/influxdb/v1.7/query_language/), with support for [bind parameters](https://docs.influxdata.com/influxdb/v1.7/tools/api/#bind-parameters) (similar to [JDBC PreparedStatement parameters](https://docs.oracle.com/javase/tutorial/jdbc/basics/prepared.html#supply_values_ps));
* it's own [QueryBuilder](https://github.com/influxdata/influxdb-java/blob/master/QUERY_BUILDER.md), as you would do with e.g. EclipseLink or Hibernate;
* Message Pack (requires InfluxDB [1.4+](https://www.influxdata.com/blog/whats-new-influxdb-oss-1-4/));
* Writing data using:
* Data Point (an object provided by this library that represents a ... data point);
* Your own POJO (you need to add a few Java Annotations);
* [InfluxDB line protocol](https://docs.influxdata.com/influxdb/v1.7/write_protocols/line_protocol_tutorial/) (for the braves only);
* UDP, as [supported by InfluxDB](https://docs.influxdata.com/influxdb/v1.7/supported_protocols/udp/);
* Support synchronous and asynchronous writes;
* Batch support configurable with `jitter` interval, `buffer` size and `flush` interval.
## Quick start
```Java
// Create an object to handle the communication with InfluxDB.
// (best practice tip: reuse the 'influxDB' instance when possible)
final String serverURL = "http://127.0.0.1:8086", username = "root", password = "root";
final InfluxDB influxDB = InfluxDBFactory.connect(serverURL, username, password);
// Create a database...
// https://docs.influxdata.com/influxdb/v1.7/query_language/database_management/
String databaseName = "NOAA_water_database";
influxDB.query(new Query("CREATE DATABASE " + databaseName));
influxDB.setDatabase(databaseName);
// ... and a retention policy, if necessary.
// https://docs.influxdata.com/influxdb/v1.7/query_language/database_management/
String retentionPolicyName = "one_day_only";
influxDB.query(new Query("CREATE RETENTION POLICY " + retentionPolicyName
+ " ON " + databaseName + " DURATION 1d REPLICATION 1 DEFAULT"));
influxDB.setRetentionPolicy(retentionPolicyName);
// Enable batch writes to get better performance.
influxDB.enableBatch(
BatchOptions.DEFAULTS
.threadFactory(runnable -> {
Thread thread = new Thread(runnable);
thread.setDaemon(true);
return thread;
})
);
// Close it if your application is terminating or you are not using it anymore.
Runtime.getRuntime().addShutdownHook(new Thread(influxDB::close));
// Write points to InfluxDB.
influxDB.write(Point.measurement("h2o_feet")
.time(System.currentTimeMillis(), TimeUnit.MILLISECONDS)
.tag("location", "santa_monica")
.addField("level description", "below 3 feet")
.addField("water_level", 2.064d)
.build());
influxDB.write(Point.measurement("h2o_feet")
.time(System.currentTimeMillis(), TimeUnit.MILLISECONDS)
.tag("location", "coyote_creek")
.addField("level description", "between 6 and 9 feet")
.addField("water_level", 8.12d)
.build());
// Wait a few seconds in order to let the InfluxDB client
// write your points asynchronously (note: you can adjust the
// internal time interval if you need via 'enableBatch' call).
Thread.sleep(5_000L);
// Query your data using InfluxQL.
// https://docs.influxdata.com/influxdb/v1.7/query_language/data_exploration/#the-basic-select-statement
QueryResult queryResult = influxDB.query(new Query("SELECT * FROM h2o_feet"));
System.out.println(queryResult);
// It will print something like:
// QueryResult [results=[Result [series=[Series [name=h2o_feet, tags=null,
// columns=[time, level description, location, water_level],
// values=[
// [2020-03-22T20:50:12.929Z, below 3 feet, santa_monica, 2.064],
// [2020-03-22T20:50:12.929Z, between 6 and 9 feet, coyote_creek, 8.12]
// ]]], error=null]], error=null]
```
## Contribute
For version change history have a look at [ChangeLog](https://github.com/influxdata/influxdb-java/blob/master/CHANGELOG.md).
### Build Requirements
* Java 1.8+
* Maven 3.5+
* Docker (for Unit testing)
Then you can build influxdb-java with all tests with:
```bash
$> export INFLUXDB_IP=127.0.0.1
$> mvn clean install
```
There is a shell script running InfluxDB and Maven from inside a Docker container and you can execute it by running:
```bash
$> ./compile-and-test.sh
```
## Useful links
* [Manual](MANUAL.md) (main documentation);
* [InfluxDB Object Mapper](INFLUXDB_MAPPER.md);
* [Query Builder](QUERY_BUILDER.md);
* [FAQ](FAQ.md);
* [Changelog](CHANGELOG.md).
## License
```license
The MIT License (MIT)
Copyright (c) 2014 Stefan Majer
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
```
| 0 |
reactor/reactor-netty | TCP/HTTP/UDP/QUIC client/server with Reactor over Netty | flux http ipc mono netty quic reactive reactive-streams reactor reactor3 tcp udp | # Reactor Netty
[](https://gitter.im/reactor/reactor-netty)
[](https://mvnrepository.com/artifact/io.projectreactor.netty/reactor-netty)
[](https://github.com/reactor/reactor-netty/actions/workflows/publish.yml) [](https://github.com/reactor/reactor-netty/actions?query=workflow%3ACodeQL)
`Reactor Netty` offers non-blocking and backpressure-ready `TCP`/`HTTP`/`UDP`/`QUIC`
clients & servers based on `Netty` framework.
## Getting it
`Reactor Netty` requires Java 8 or + to run.
With `Gradle` from [repo.spring.io](https://repo.spring.io) or `Maven Central` repositories (stable releases only):
```groovy
repositories {
//maven { url 'https://repo.spring.io/snapshot' }
maven { url 'https://repo.spring.io/milestone' }
mavenCentral()
}
dependencies {
//compile "io.projectreactor.netty:reactor-netty-core:1.2.0-SNAPSHOT"
compile "io.projectreactor.netty:reactor-netty-core:1.2.0-M1"
//compile "io.projectreactor.netty:reactor-netty-http:1.2.0-SNAPSHOT"
compile "io.projectreactor.netty:reactor-netty-http:1.2.0-M1"
}
```
See the [Reference documentation](https://projectreactor.io/docs/netty/release/reference/index.html#getting)
for more information on getting it (eg. using `Maven`, or on how to get milestones and snapshots).
## Getting Started
New to `Reactor Netty`? Check this [Reactor Netty Workshop](https://violetagg.github.io/reactor-netty-workshop/)
and the [Reference documentation](https://projectreactor.io/docs/netty/release/reference/index.html)
Here is a very simple `HTTP` server and the corresponding `HTTP` client example
```java
HttpServer.create() // Prepares an HTTP server ready for configuration
.port(0) // Configures the port number as zero, this will let the system pick up
// an ephemeral port when binding the server
.route(routes ->
// The server will respond only on POST requests
// where the path starts with /test and then there is path parameter
routes.post("/test/{param}", (request, response) ->
response.sendString(request.receive()
.asString()
.map(s -> s + ' ' + request.param("param") + '!')
.log("http-server"))))
.bindNow(); // Starts the server in a blocking fashion, and waits for it to finish its initialization
```
```java
HttpClient.create() // Prepares an HTTP client ready for configuration
.port(server.port()) // Obtains the server's port and provides it as a port to which this
// client should connect
.post() // Specifies that POST method will be used
.uri("/test/World") // Specifies the path
.send(ByteBufFlux.fromString(Flux.just("Hello"))) // Sends the request body
.responseContent() // Receives the response body
.aggregate()
.asString()
.log("http-client")
.block();
```
## Getting help
Having trouble with `Reactor Netty`? We'd like to help!
* If you are upgrading, read the [release notes](https://github.com/reactor/reactor-netty/releases)
for upgrade instructions and *new and noteworthy* features.
* Ask a question - we monitor [stackoverflow.com](https://stackoverflow.com) for questions
tagged with [`reactor-netty`](https://stackoverflow.com/questions/tagged/reactor-netty). You can also chat
with the community on [Gitter](https://gitter.im/reactor/reactor-netty).
* Report bugs with `Reactor Netty` at [github.com/reactor/reactor-netty/issues](https://github.com/reactor/reactor-netty/issues).
* More about [Support and Deprecation policies](https://github.com/reactor/.github/blob/main/SUPPORT.adoc)
## Reporting Issues
`Reactor Netty` uses `GitHub’s` integrated issue tracking system to record bugs and feature requests.
If you want to raise an issue, please follow the recommendations below:
* Before you log a bug, please [search the issue tracker](https://github.com/reactor/reactor-netty/search?type=Issues)
to see if someone has already reported the problem.
* If the issue doesn't already exist, [create a new issue](https://github.com/reactor/reactor-netty/issues/new/choose).
* Please provide as much information as possible with the issue report, we like to know
the version of `Reactor Netty` that you are using, as well as your `Operating System` and
`JVM` version.
* If you want to raise a security vulnerability, please review our [Security Policy](https://github.com/reactor/reactor-netty/security/policy) for more details.
## Contributing
See our [Contributing Guide](https://github.com/reactor/.github/blob/main/CONTRIBUTING.md) for information about contributing to `Reactor Netty`.
## Building from Source
You don't need to build from source to use `Reactor Netty` (binaries in
[repo.spring.io](https://repo.spring.io)), but if you want to try out the latest and
greatest, `Reactor Netty` can be easily built with the
[gradle wrapper](https://docs.gradle.org/current/userguide/gradle_wrapper.html). You also need JDK 1.8.
```shell
$ git clone https://github.com/reactor/reactor-netty.git
$ cd reactor-netty
$ ./gradlew build
```
If you want to publish the artifacts to your local `Maven` repository use:
```shell
$ ./gradlew publishToMavenLocal
```
## Javadoc
https://projectreactor.io/docs/netty/release/api/
## Guides
* https://projectreactor.io/docs/netty/release/reference/index.html
* https://violetagg.github.io/reactor-netty-workshop/
## License
`Reactor Netty` is Open Source Software released under the [Apache License 2.0](https://www.apache.org/licenses/LICENSE-2.0)
| 0 |
apache/nifi | Apache NiFi | hacktoberfest java nifi | <!--
Licensed to the Apache Software Foundation (ASF) under one or more
contributor license agreements. See the NOTICE file distributed with
this work for additional information regarding copyright ownership.
The ASF licenses this file to You under the Apache License, Version 2.0
(the "License"); you may not use this file except in compliance with
the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-->
[<img src="https://nifi.apache.org/assets/images/apache-nifi-logo.svg" width="300" height="126" alt="Apache NiFi"/>][nifi]
[](https://github.com/apache/nifi/actions/workflows/ci-workflow.yml)
[](https://github.com/apache/nifi/actions/workflows/system-tests.yml)
[](https://github.com/apache/nifi/actions/workflows/integration-tests.yml)
[](https://github.com/apache/nifi/actions/workflows/docker-tests.yml)
[](https://github.com/apache/nifi/actions/workflows/dependency-check.yml)
[](https://codecov.io/gh/apache/nifi)
[](https://hub.docker.com/r/apache/nifi/)
[](https://nifi.apache.org/download.html)
[](https://s.apache.org/nifi-community-slack)
[Apache NiFi](https://nifi.apache.org/) is an easy to use, powerful, and
reliable system to process and distribute data.
## Table of Contents
- [Features](#features)
- [Requirements](#requirements)
- [Getting Started](#getting-started)
- [MiNiFi subproject](#minifi-subproject)
- [Registry subproject](#registry-subproject)
- [Getting Help](#getting-help)
- [Documentation](#documentation)
- [License](#license)
- [Export Control](#export-control)
## Features
Apache NiFi was made for dataflow. It supports highly configurable directed graphs of data routing, transformation, and system mediation logic. Some of its key features include:
- Web-based user interface
- Seamless experience for design, control, and monitoring
- Multi-tenant user experience
- Highly configurable
- Loss tolerant vs guaranteed delivery
- Low latency vs high throughput
- Dynamic prioritization
- Flows can be modified at runtime
- Back pressure
- Scales up to leverage full machine capability
- Scales out with zero-leader clustering model
- Data Provenance
- Track dataflow from beginning to end
- Designed for extension
- Build your own processors and more
- Enables rapid development and effective testing
- Secure
- SSL, SSH, HTTPS, encrypted content, etc...
- Pluggable fine-grained role-based authentication/authorization
- Multiple teams can manage and share specific portions of the flow
## Minimum Recommendations
* JDK 21
* Apache Maven 3.9.2
## Minimum Requirements
* JDK 21
* Apache Maven 3.9.2
## Getting Started
Read through the [quickstart guide for development](https://nifi.apache.org/quickstart.html).
It will include information on getting a local copy of the source, give pointers on issue
tracking, and provide some warnings about common problems with development environments.
For a more comprehensive guide to development and information about contributing to the project
read through the [NiFi Developer's Guide](https://nifi.apache.org/developer-guide.html).
### Building
Run the following Maven command to build standard project modules using parallel execution:
./mvnw clean install -T2C
Run the following Maven command to build project modules with static analysis to confirm compliance with code and licensing requirements:
./mvnw clean install -T2C -P contrib-check
Building on Microsoft Windows requires using `mvnw.cmd` instead of `mwnw` to run the Maven Wrapper.
### Deploying
Change directories to `nifi-assembly`. The `target` directory contains binary archives.
laptop:nifi myuser$ cd nifi-assembly
laptop:nifi-assembly myuser$ ls -lhd target/nifi*
drwxr-xr-x 3 myuser mygroup 102B Apr 30 00:29 target/nifi-1.0.0-SNAPSHOT-bin
-rw-r--r-- 1 myuser mygroup 144M Apr 30 00:30 target/nifi-1.0.0-SNAPSHOT-bin.tar.gz
-rw-r--r-- 1 myuser mygroup 144M Apr 30 00:30 target/nifi-1.0.0-SNAPSHOT-bin.zip
Copy the `nifi-VERSION-bin.tar.gz` or `nifi-VERSION-bin.zip` to a separate deployment directory.
Extracting the distribution will create a new directory named for the version.
laptop:nifi-assembly myuser$ mkdir ~/example-nifi-deploy
laptop:nifi-assembly myuser$ tar xzf target/nifi-*-bin.tar.gz -C ~/example-nifi-deploy
laptop:nifi-assembly myuser$ ls -lh ~/example-nifi-deploy/
total 0
drwxr-xr-x 10 myuser mygroup 340B Apr 30 01:06 nifi-1.0.0-SNAPSHOT
### Starting
Change directories to the deployment location and run the following command to start NiFi.
laptop:~ myuser$ cd ~/example-nifi-deploy/nifi-*
laptop:nifi-1.0.0-SNAPSHOT myuser$ ./bin/nifi.sh start
Running `bin/nifi.sh start` starts NiFi in the background and exits. Use `--wait-for-init` with an optional timeout in
seconds to wait for a complete startup before exiting.
laptop:nifi-1.0.0-SNAPSHOT myuser$ ./bin/nifi.sh start --wait-for-init 120
### Authenticating
The default configuration generates a random username and password on startup. NiFi writes the generated credentials
to the application log located in `logs/nifi-app.log` under the NiFi installation directory.
The following command can be used to find the generated credentials on operating systems with `grep` installed:
laptop:nifi-1.0.0-SNAPSHOT myuser$ grep Generated logs/nifi-app*log
NiFi logs the generated credentials as follows:
Generated Username [USERNAME]
Generated Password [PASSWORD]
The `USERNAME` will be a random UUID composed of 36 characters. The `PASSWORD` will be a random string composed of
32 characters. The generated credentials will be stored in `conf/login-identity-providers.xml` with the password stored
using bcrypt hashing. Record these credentials in a secure location for access to NiFi.
The random username and password can be replaced with custom credentials using the following command:
./bin/nifi.sh set-single-user-credentials <username> <password>
### Running
Open the following link in a web browser to access NiFi: https://localhost:8443/nifi
The web browser will display a warning message indicating a potential security risk due to the self-signed
certificate NiFi generated during initialization. Accepting the potential security risk and continuing to load
the interface is an option for initial development installations. Production deployments should provision a certificate
from a trusted certificate authority and update the NiFi keystore and truststore configuration.
Accessing NiFi after accepting the self-signed certificate will display the login screen.

Using the generated credentials, enter the generated username in the `User` field
and the generated password in the `Password` field, then select `LOG IN` to access the system.

### Configuring
The [NiFi User Guide](https://nifi.apache.org/docs/nifi-docs/html/user-guide.html) describes how to build a data flow.
### Stopping
Run the following command to stop NiFi:
laptop:~ myuser$ cd ~/example-nifi-deploy/nifi-*
laptop:nifi-1.0.0-SNAPSHOT myuser$ ./bin/nifi.sh stop
## MiNiFi subproject
MiNiFi is a child project effort of Apache NiFi. It is a complementary data collection approach that supplements the core tenets of [NiFi](https://nifi.apache.org/) in dataflow management, focusing on the collection of data at the source of its creation.
Specific goals for MiNiFi are comprised of:
- small and lightweight footprint
- central management of agents
- generation of data provenance
- integration with NiFi for follow-on dataflow management and full chain of custody of information
Perspectives of the role of MiNiFi should be from the perspective of the agent acting immediately at, or directly adjacent to, source sensors, systems, or servers.
To run:
- Change directory to 'minifi-assembly'. In the target directory, there should be a build of minifi.
$ cd minifi-assembly
$ ls -lhd target/minifi*
drwxr-xr-x 3 user staff 102B Jul 6 13:07 minifi-1.14.0-SNAPSHOT-bin
-rw-r--r-- 1 user staff 39M Jul 6 13:07 minifi-1.14.0-SNAPSHOT-bin.tar.gz
-rw-r--r-- 1 user staff 39M Jul 6 13:07 minifi-1.14.0-SNAPSHOT-bin.zip
- For testing ongoing development you could use the already unpacked build present in the directory
named "minifi-*version*-bin", where *version* is the current project version. To deploy in another
location make use of either the tarball or zipfile and unpack them wherever you like. The
distribution will be within a common parent directory named for the version.
$ mkdir ~/example-minifi-deploy
$ tar xzf target/minifi-*-bin.tar.gz -C ~/example-minifi-deploy
$ ls -lh ~/example-minifi-deploy/
total 0
drwxr-xr-x 10 user staff 340B Jul 6 01:06 minifi-1.14.0-SNAPSHOT
To run MiNiFi:
- Change directory to the location where you installed MiNiFi and run it.
$ cd ~/example-minifi-deploy/minifi-*
$ ./bin/minifi.sh start
- View the logs located in the logs folder
$ tail -F ~/example-minifi-deploy/logs/minifi-app.log
- For help building your first data flow and sending data to a NiFi instance see the System Admin Guide located in the docs folder or making use of the minifi-toolkit.
- If you are testing ongoing development, you will likely want to stop your instance.
$ cd ~/example-minifi-deploy/minifi-*
$ ./bin/minifi.sh stop
### Docker Build
To build:
- Run a full NiFi build (see above for instructions). Then from the minifi/ subdirectory, execute `mvn -P docker clean install`. This will run the full build, create a docker image based on it, and run docker-compose integration tests. After it completes successfully, you should have an apacheminifi:${minifi.version} image that can be started with the following command (replacing ${minifi.version} with the current maven version of your branch):
```
docker run -d -v YOUR_CONFIG.YML:/opt/minifi/minifi-${minifi.version}/conf/config.yml apacheminifi:${minifi.version}
```
## Registry subproject
Registry—a subproject of Apache NiFi—is a complementary application that provides a central location for storage and management of shared resources across one or more instances of NiFi and/or MiNiFi.
### Getting Registry Started
1) Build NiFi (see [Getting Started for NiFi](#getting-started) )
or
Build only the Registry subproject:
cd nifi/nifi-registry
mvn clean install
If you wish to enable style and license checks, specify the contrib-check profile:
mvn clean install -Pcontrib-check
2) Start Registry
cd nifi-registry/nifi-registry-assembly/target/nifi-registry-<VERSION>-bin/nifi-registry-<VERSION>/
./bin/nifi-registry.sh start
Note that the application web server can take a while to load before it is accessible.
3) Accessing the application web UI
With the default settings, the application UI will be available at [http://localhost:18080/nifi-registry](http://localhost:18080/nifi-registry)
4) Accessing the application REST API
If you wish to test against the application REST API, you can access the REST API directly. With the default settings, the base URL of the REST API will be at `http://localhost:18080/nifi-registry-api`. A UI for testing the REST API will be available at [http://localhost:18080/nifi-registry-api/swagger/ui.html](http://localhost:18080/nifi-registry-api/swagger/ui.html)
5) Accessing the application logs
Logs will be available in `logs/nifi-registry-app.log`
### Database Testing
In order to ensure that NiFi Registry works correctly against different relational databases,
the existing integration tests can be run against different databases by leveraging the [Testcontainers framework](https://www.testcontainers.org/).
Spring profiles are used to control the DataSource factory that will be made available to the Spring application context.
DataSource factories are provided that use the Testcontainers framework to start a Docker container for a given database and create a corresponding DataSource.
If no profile is specified then an H2 DataSource will be used by default and no Docker containers are required.
Assuming Docker is running on the system where the build is running, then the following commands can be run:
| Target Database | Build Command |
|-----------------|--------------------------------------------------------------------|
| All supported | `mvn verify -Ptest-all-dbs` |
| H2 (default) | `mvn verify` |
| MariaDB 10.3 | `mvn verify -Pcontrib-check -Dspring.profiles.active=mariadb-10-3` |
| MySQL 8 | `mvn verify -Pcontrib-check -Dspring.profiles.active=mysql-8` |
| PostgreSQL 10 | `mvn verify -Dspring.profiles.active=postgres-10` |
For a full list of the available DataSource factories, consult the `nifi-registry-test` module.
## Getting Help
If you have questions, you can reach out to our mailing list: dev@nifi.apache.org
([archive](https://lists.apache.org/list.html?dev@nifi.apache.org)). For more interactive discussions, community members can often be found in the following locations:
- Apache NiFi Slack Workspace: https://apachenifi.slack.com/
New users can join the workspace using the following [invite link](https://join.slack.com/t/apachenifi/shared_invite/zt-11njbtkdx-ZRU8FKYSWoEHRJetidy0zA).
To submit a feature request or bug report, please file a Jira at [https://issues.apache.org/jira/projects/NIFI/issues](https://issues.apache.org/jira/projects/NIFI/issues). If this is a **security vulnerability report**, please email [security@nifi.apache.org](mailto:security@nifi.apache.org) directly and review the [Apache NiFi Security Vulnerability Disclosure](https://nifi.apache.org/security.html) and [Apache Software Foundation Security](https://www.apache.org/security/committers.html) processes first.
## Documentation
See https://nifi.apache.org/ for the latest NiFi documentation.
See https://nifi.apache.org/minifi and https://cwiki.apache.org/confluence/display/MINIFI for the latest MiNiFi-specific documentation.
See https://nifi.apache.org/registry for the latest Registry-specific documentation.
## License
Except as otherwise noted this software is licensed under the
[Apache License, Version 2.0](https://www.apache.org/licenses/LICENSE-2.0.html)
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
https://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
## Export Control
This distribution includes cryptographic software. The country in which you
currently reside may have restrictions on the import, possession, use, and/or
re-export to another country, of encryption software. BEFORE using any
encryption software, please check your country's laws, regulations and
policies concerning the import, possession, or use, and re-export of encryption
software, to see if this is permitted. See <https://www.wassenaar.org/> for more
information.
The U.S. Government Department of Commerce, Bureau of Industry and Security
(BIS), has classified this software as Export Commodity Control Number (ECCN)
5D002.C.1, which includes information security software using or performing
cryptographic functions with asymmetric algorithms. The form and manner of this
Apache Software Foundation distribution makes it eligible for export under the
License Exception ENC Technology Software Unrestricted (TSU) exception (see the
BIS Export Administration Regulations, Section 740.13) for both object code and
source code.
The following provides more details on the included cryptographic software:
Apache NiFi uses BouncyCastle, JCraft Inc., and the built-in
Java cryptography libraries for SSL, SSH, and the protection
of sensitive configuration parameters. See
- https://bouncycastle.org/about.html
- http://www.jcraft.com/c-info.html
- https://www.oracle.com/corporate/security-practices/corporate/governance/global-trade-compliance.html
for more details on each of these libraries cryptography features.
[nifi]: https://nifi.apache.org/
[logo]: https://nifi.apache.org/assets/images/apache-nifi-logo.svg
| 0 |
actiontech/dble | A High Scalability Middle-ware for MySQL Sharding | dble distributed-database mysql mysql-sharding sharding | 
[](https://github.com/actiontech/dble/issues)
[](https://github.com/actiontech/dble/issues?q=is%3Aissue+is%3Aclosed)
[](https://github.com/actiontech/dble/network/members)
[](https://github.com/actiontech/dble/stargazers)
[](https://github.com/actiontech/dble/graphs/contributors)
[](https://github.com/actiontech/dble/blob/master/LICENSE)
[](https://travis-ci.com/actiontech?tab=insights)
[](https://github.com/actiontech/dble/releases)
[](https://github.com/actiontech/dble/releases)
[](https://github.com/actiontech/dble)
dble (pronouced "double", less bug and no "ou") is maintained by [ActionTech](https://opensource.actionsky.com).
DBLE由上海爱可生信息技术股份有限公司(以下简称爱可生公司)出品和维护。
DBLE是爱可生公司“云树分布式数据库软件(简称‘云树®Shard’或‘CTREE Shard’)”软件产品的开源版本。
## What is dble?
dble is a high scalability middle-ware for MySQL sharding.
- __Sharding__
As your business grows, you can use dble to replace the origin single MySQL instance.
- __Compatible with MySQL protocol__
Use dble as same as MySQL. You can replace MySQL with dble to power your application without changing a single line of code in most cases.
- __High availability__
dble server can be used as clustered, business will not suffer from single node fail.
- __SQL Support__
Support(some in Roadmap) SQL 92 standard and MySQL dialect. We support complex SQL query like group by, order by, distinct, join ,union, sub-query(in Roadmap) and so on.
- __Complex Query Optimization__
Optimize the complex query, including, without limitation, Global-table join sharding-table, ER-relation tables, Sub-Queries, Simplifying select items, and the like.
- __Distributed Transaction__
Support Distributed Transaction using two-phase commit. You can choose normal mode for performance or XA mode for data safety, of course, the XA mode dependent on MySQL-5.7's XA Transaction, MySQL node's high availability and data reliability of disk.
## History
dble is based on [MyCat](https://github.com/MyCATApache/Mycat-Server). First of all, thanks to the contributors from MyCat.
For us, focusing on MySQL is a better choice. So we cancelled the support for other databases, deeply improved/optimized its behavior on compatibility, complex query and distributed transaction. And of course, fixed lots of bugs.
For more details, see [Roadmap](./docs/ROADMAP.md) and [Issues](https://github.com/actiontech/dble/issues) .
## Roadmap
Read the [Roadmap](./docs/ROADMAP.md).
## Architecture

## Quick start
Read the [Quick Start](./docs/QUICKSTART.md) or [Quick Start With Docker](./docs/dble_quick_start_docker.md) or [Quick Start With Docker-Compose](./docs/dble_start_docker_compose.md).
参见文档[快速开始](https://github.com/actiontech/dble-docs-cn/blob/master/0.overview/0.3_dble_quick_start.md)或者[Docker快速开始](https://github.com/actiontech/dble-docs-cn/blob/master/0.overview/0.4_dble_quick_start_docker.md)或者[Docker-Compose快速开始](https://github.com/actiontech/dble-docs-cn/blob/master/0.overview/0.5_dble_start_docker_compose.md).
## Official website
For more information, please visit the [official website](https://opensource.actionsky.com).
## Download
[Releases Download Link](https://github.com/actiontech/dble/releases) Or [The Other Image](https://hub.fastgit.org/actiontech/dble/releases)
## Documentation
+ [简体中文](https://actiontech.github.io/dble-docs-cn/)
+ 最新PDF版本请去[文档PDF下载](https://github.com/actiontech/dble-docs-cn/releases/download/3.22.11.0%2Ftag/dble-manual-3.22.11.0.pdf) 或者[github镜像站下载](https://hub.fastgit.org/actiontech/dble-docs-cn/releases/download/3.22.11.0%2Ftag/dble-manual-3.22.11.0.pdf) 或者去[文档中心](https://actiontech.github.io/dble-docs-cn/)选择合适的版本下载
+ [中文公开课](https://opensource.actionsky.com/dble-lessons/)
+ English(Comming soon)
## Contributing
Contributions are welcomed and greatly appreciated. See [CONTRIBUTING.md](./docs/CONTRIBUTING.md)
for details on submitting patches and the contribution workflow.
## Community
* IRC: [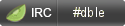](https://kiwiirc.com/client/irc.freenode.net/?nick=user|?&theme=cli#dble)
* QQ group: 669663113
* [If you're using DBLE, please let us know.](https://wj.qq.com/s/2291106/09f4)
* wechat subscription QR code

## Contact us
Dble has enterprise support plans, you may contact our sales team:
* Global Sales: 400-820-6580
* North China: 86-13910506562, Mr.Wang
* South China: 86-18503063188, Mr.Cao
* East China: 86-18930110869, Mr.Liang
* South-West China: 86-13540040119, Mr.Hong
| 0 |
JoyChou93/java-sec-code | Java web common vulnerabilities and security code which is base on springboot and spring security | benchmark code cors deserialize java jsonp rce rmi security spel sqli ssrf tomcat web xxe | # Java Sec Code
Java sec code is a very powerful and friendly project for learning Java vulnerability code.
[中文文档](https://github.com/JoyChou93/java-sec-code/blob/master/README_zh.md) 😋
## Introduce
This project can also be called Java vulnerability code.
Each vulnerability type code has a security vulnerability by default unless there is no vulnerability. The relevant fix code is in the comments or code. Specifically, you can view each vulnerability code and comments.
Due to the server expiration, the online demo site had to go offline.
Login username & password:
```
admin/admin123
joychou/joychou123
```
## Vulnerability Code
Sort by letter.
- [Actuators to RCE](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/resources/logback-online.xml)
- [CommandInject](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/CommandInject.java)
- [CORS](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/CORS.java)
- [CRLF Injection](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/CRLFInjection.java)
- [CSRF](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/security/WebSecurityConfig.java)
- [CVE-2022-22978](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/security/WebSecurityConfig.java)
- [Deserialize](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/Deserialize.java)
- [Fastjson](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/Fastjson.java)
- [File Upload](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/FileUpload.java)
- [GetRequestURI](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/GetRequestURI.java)
- [IP Forge](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/IPForge.java)
- [Java RMI](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/RMI/Server.java)
- [JSONP](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/Jsonp.java)
- [Log4j](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/Log4j.java)
- [ooxmlXXE](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/othervulns/ooxmlXXE.java)
- [PathTraversal](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/PathTraversal.java)
- [QLExpress](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/QLExpress.java)
- [RCE](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/Rce.java)
- Runtime
- ProcessBuilder
- ScriptEngine
- Yaml Deserialize
- Groovy
- [Shiro](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/Shiro.java)
- [Swagger](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/config/SwaggerConfig.java)
- [SpEL](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/SpEL.java)
- [SQL Injection](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/SQLI.java)
- [SSRF](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/SSRF.java)
- [SSTI](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/SSTI.java)
- [URL Redirect](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/URLRedirect.java)
- [URL whitelist Bypass](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/URLWhiteList.java)
- [xlsxStreamerXXE](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/othervulns/xlsxStreamerXXE.java)
- [XSS](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/XSS.java)
- [XStream](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/XStreamRce.java)
- [XXE](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/XXE.java)
- [JWT](https://github.com/JoyChou93/java-sec-code/blob/master/src/main/java/org/joychou/controller/Jwt.java)
## Vulnerability Description
- [Actuators to RCE](https://github.com/JoyChou93/java-sec-code/wiki/Actuators-to-RCE)
- [CORS](https://github.com/JoyChou93/java-sec-code/wiki/CORS)
- [CSRF](https://github.com/JoyChou93/java-sec-code/wiki/CSRF)
- [Deserialize](https://github.com/JoyChou93/java-sec-code/wiki/Deserialize)
- [Fastjson](https://github.com/JoyChou93/java-sec-code/wiki/Fastjson)
- [Java RMI](https://github.com/JoyChou93/java-sec-code/wiki/Java-RMI)
- [JSONP](https://github.com/JoyChou93/java-sec-code/wiki/JSONP)
- [POI-OOXML XXE](https://github.com/JoyChou93/java-sec-code/wiki/Poi-ooxml-XXE)
- [SQLI](https://github.com/JoyChou93/java-sec-code/wiki/SQL-Inject)
- [SSRF](https://github.com/JoyChou93/java-sec-code/wiki/SSRF)
- [SSTI](https://github.com/JoyChou93/java-sec-code/wiki/SSTI)
- [URL whitelist Bypass](https://github.com/JoyChou93/java-sec-code/wiki/URL-whtielist-Bypass)
- [XXE](https://github.com/JoyChou93/java-sec-code/wiki/XXE)
- [JWT](https://github.com/JoyChou93/java-sec-code/wiki/JWT)
- [Others](https://github.com/JoyChou93/java-sec-code/wiki/others)
## How to run
The application will use mybatis auto-injection. Please run mysql server ahead of time and configure the mysql server database's name and username/password except docker environment.
```
spring.datasource.url=jdbc:mysql://127.0.0.1:3306/java_sec_code
spring.datasource.username=root
spring.datasource.password=woshishujukumima
```
- Docker
- IDEA
- Tomcat
- JAR
### Docker
Start docker:
```
docker-compose pull
docker-compose up
```
Stop docker:
```
docker-compose down
```
Docker's environment:
- Java 1.8.0_102
- Mysql 8.0.17
- Tomcat 8.5.11
### IDEA
- `git clone https://github.com/JoyChou93/java-sec-code`
- Open in IDEA and click `run` button.
Example:
```
http://localhost:8080/rce/exec?cmd=whoami
```
return:
```
Viarus
```
### Tomcat
- `git clone https://github.com/JoyChou93/java-sec-code` & `cd java-sec-code`
- Build war package by `mvn clean package`.
- Copy war package to tomcat webapps directory.
- Start tomcat application.
Example:
```
http://localhost:8080/java-sec-code-1.0.0/rce/runtime/exec?cmd=whoami
```
return:
```
Viarus
```
### JAR
Change `war` to `jar` in `pom.xml`.
```xml
<groupId>sec</groupId>
<artifactId>java-sec-code</artifactId>
<version>1.0.0</version>
<packaging>war</packaging>
```
Build package and run.
```
git clone https://github.com/JoyChou93/java-sec-code
cd java-sec-code
mvn clean package -DskipTests
java -jar target/java-sec-code-1.0.0.jar
```
## Authenticate
### Login
[http://localhost:8080/login](http://localhost:8080/login)
If you are not logged in, accessing any page will redirect you to the login page. The username & password are as follows.
```
admin/admin123
joychou/joychou123
```
### Logout
[http://localhost:8080/logout](http://localhost:8080/logout)
### RememberMe
Tomcat's default JSESSION session is valid for 30 minutes, so a 30-minute non-operational session will expire. In order to solve this problem, the rememberMe function is introduced, and the default expiration time is 2 weeks.
## Contributors
Core developers : [JoyChou](https://github.com/JoyChou93), [liergou9981](https://github.com/liergou9981)
Other developers: [lightless](https://github.com/lightless233), [Anemone95](https://github.com/Anemone95), [waderwu](https://github.com/waderwu).
## Donate
If you like the poject, you can donate to support me. With your support, I will be able to make `Java sec code` better 😎.
### Alipay
Scan the QRcode to support `Java sec code`.
<img title="Alipay QRcode" src="https://aliyun-testaaa.oss-cn-shanghai.aliyuncs.com/alipay_qr.png" width="200">
| 0 |
apache/cloudstack | Apache CloudStack is an opensource Infrastructure as a Service (IaaS) cloud computing platform | cloud cloudstack iaas infrastructure java kubernetes kvm libvirt orchestration python virtual-machine virtualization vmware vsphere xcp-ng xenserver | # Apache CloudStack [](https://github.com/apache/cloudstack/actions/workflows/build.yml) [](https://github.com/apache/cloudstack/actions/workflows/ui.yml) [](https://github.com/apache/cloudstack/actions/workflows/rat.yml) [](https://github.com/apache/cloudstack/actions/workflows/ci.yml) [](https://sonarcloud.io/dashboard?id=apache_cloudstack) [](https://codecov.io/gh/apache/cloudstack)
[](https://cloudstack.apache.org/)
Apache CloudStack is open source software designed to deploy and manage large
networks of virtual machines, as a highly available, highly scalable
Infrastructure as a Service (IaaS) cloud computing platform. CloudStack is used
by a number of service providers to offer public cloud services, and by many
companies to provide an on-premises (private) cloud offering, or as part of a
hybrid cloud solution.
CloudStack is a turnkey solution that includes the entire "stack" of features
most organizations want with an IaaS cloud: compute orchestration,
Network-as-a-Service, user and account management, a full and open native API,
resource accounting, and a first-class User Interface (UI).
CloudStack currently supports the most popular hypervisors:
VMware vSphere, KVM, XenServer, XenProject and Hyper-V as well as
OVM and LXC containers.
Users can manage their cloud with an easy to use Web interface, command line
tools, and/or a full-featured query based API.
For more information on Apache CloudStack, please visit the [website](http://cloudstack.apache.org)
## Who Uses CloudStack?
* There are more than 150 known organizations using Apache CloudStack (or a commercial distribution of CloudStack). Our users include many major service providers running CloudStack to offer public cloud services, product vendors who incorporate or integrate with CloudStack in their own products, organizations who have used CloudStack to build their own private clouds, and systems integrators that offer CloudStack related services.
* See our [case studies](https://cwiki.apache.org/confluence/display/CLOUDSTACK/Case+Studies) highlighting successful deployments of Apache CloudStack.
* See the up-to-date list of current [users](https://cloudstack.apache.org/users.html).
* If you are using CloudStack in your organization and your company is not listed above, please complete our brief adoption [survey](https://cloudstack.apache.org/survey.html). We're happy to keep your company name anonymous if you require.
## Demo

See the project user-interface QA website that runs CloudStack against simulator hypervisor:
https://qa.cloudstack.cloud/simulator/ (admin:password)
## Getting Started
* Download a released [version](https://cloudstack.apache.org/downloads.html)
* Build from source with the instructions in the [INSTALL.md](INSTALL.md) file.
## Getting Source Repository
Apache CloudStack project uses Git. The official Git repository is at:
https://gitbox.apache.org/repos/asf/cloudstack.git
And a mirror is hosted on GitHub:
https://github.com/apache/cloudstack
The GitHub mirror is strictly read only and provides convenience to users and
developers to explore the code and for the community to accept contributions
via GitHub pull requests.
## Documentation
* [Project Documentation](https://docs.cloudstack.apache.org)
* [Release notes](https://docs.cloudstack.apache.org/en/latest/releasenotes/index.html)
* Developer [wiki](https://cwiki.apache.org/confluence/display/CLOUDSTACK/Home)
* Design [documents](https://cwiki.apache.org/confluence/display/CLOUDSTACK/Design)
* API [documentation](https://cloudstack.apache.org/api.html)
* How to [contribute](CONTRIBUTING.md)
## News and Events
* [Blog](https://blogs.apache.org/cloudstack)
* [Twitter](https://twitter.com/cloudstack)
* [Events and meetup](http://cloudstackcollab.org/)
* [YouTube channel](https://www.youtube.com/ApacheCloudStack)
## Getting Involved and Contributing
Interested in helping out with Apache CloudStack? Great! We welcome
participation from anybody willing to work [The Apache Way](http://theapacheway.com) and make a
contribution. Note that you do not have to be a developer in order to contribute
to Apache CloudStack. We need folks to help with documentation, translation,
promotion etc. See our contribution [page](http://cloudstack.apache.org/contribute.html).
If you are a frequent contributors, you can request to be added as collaborators
(see https://cwiki.apache.org/confluence/display/INFRA/Git+-+.asf.yaml+features#Git.asf.yamlfeatures-AssigningexternalcollaboratorswiththetriageroleonGitHub)
to our GitHub repos. This allows you to use project GitHub with ability to report
issue with tags, and be assigned to issues and PRs. This is done via the .asf.yaml
file in this repo.
You may do so by sharing your GitHub users ID or raise a GitHub issue.
If you're interested in learning more or participating in the Apache CloudStack
project, the mailing lists are the best way to do that. While the project has
several communications channels, the [mailing lists](http://cloudstack.apache.org/mailing-lists.html) are the most active and the
official channels for making decisions about the project itself.
Mailing lists:
- [Development Mailing List](mailto:dev-subscribe@cloudstack.apache.org)
- [Users Mailing List](mailto:users-subscribe@cloudstack.apache.org)
- [Commits Mailing List](mailto:commits-subscribe@cloudstack.apache.org)
- [Issues Mailing List](mailto:issues-subscribe@cloudstack.apache.org)
- [Marketing Mailing List](mailto:marketing-subscribe@cloudstack.apache.org)
Report and/or check bugs on [GitHub](https://github.com/apache/cloudstack/issues) and check our
developer [page](https://cloudstack.apache.org/developers.html) for contributing code.
## Reporting Security Vulnerabilities
If you've found an issue that you believe is a security vulnerability in a
released version of CloudStack, please report it to `security@apache.org` with
details about the vulnerability, how it might be exploited, and any additional
information that might be useful.
For more details, please visit our security [page](http://cloudstack.apache.org/security.html).
## License
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
Please see the [LICENSE](LICENSE) file included in the root directory
of the source tree for extended license details.
## Notice of Cryptographic Software
This distribution includes cryptographic software. The country in which you currently
reside may have restrictions on the import, possession, use, and/or re-export to another
country, of encryption software. BEFORE using any encryption software, please check your
country's laws, regulations and policies concerning the import, possession, or use, and
re-export of encryption software, to see if this is permitted. See [The Wassenaar Arrangement](http://www.wassenaar.org/)
for more information.
The U.S. Government Department of Commerce, Bureau of Industry and Security (BIS), has
classified this software as Export Commodity Control Number (ECCN) 5D002.C.1, which
includes information security software using or performing cryptographic functions with
asymmetric algorithms. The form and manner of this Apache Software Foundation distribution
makes it eligible for export under the License Exception ENC Technology Software
Unrestricted (TSU) exception (see the BIS Export Administration Regulations, Section
740.13) for both object code and source code.
The following provides more details on the included cryptographic software:
* CloudStack makes use of JaSypt cryptographic libraries.
* CloudStack has a system requirement of MySQL, and uses native database encryption functionality.
* CloudStack makes use of the Bouncy Castle general-purpose encryption library.
* CloudStack can optionally interact with and control OpenSwan-based VPNs.
* CloudStack has a dependency on and makes use of JSch - a java SSH2 implementation.
| 0 |
hazelcast/hazelcast | Hazelcast is a unified real-time data platform combining stream processing with a fast data store, allowing customers to act instantly on data-in-motion for real-time insights. | big-data caching data-in-motion data-insights distributed distributed-computing distributed-systems hacktoberfest hazelcast in-memory java low-latency real-time scalability stream-processing | # Hazelcast
[](https://slack.hazelcast.com/)
[](https://javadoc.io/doc/com.hazelcast/hazelcast/latest)
[](https://hub.docker.com/r/hazelcast/hazelcast)
[](https://sonarcloud.io/dashboard?id=hazelcast_hazelcast)
----
## What is Hazelcast
The world’s leading companies trust Hazelcast to modernize applications and take instant action on data in motion to create new revenue streams, mitigate risk, and operate more efficiently. Businesses use Hazelcast’s unified **real-time data platform** to process **streaming** data, enrich it with historical context and take instant action with standard or **ML/AI-driven automation** - before it is stored in a database or data lake.
Hazelcast is named in the Gartner Market Guide to Event Stream Processing and a leader in the GigaOm Radar Report for Streaming Data Platforms. To join our community of CXOs, architects and developers at brands such as Lowe’s, HSBC, JPMorgan Chase, Volvo, New York Life, and others, visit [hazelcast.com](https://hazelcast.com).
## When to use Hazelcast
Hazelcast provides a platform that can handle multiple types of workloads for
building real-time applications.
* Stateful data processing over streaming data or data at rest
* Querying streaming and batch data sources directly using SQL
* Ingesting data through a library of connectors and serving it using
low-latency SQL queries
* Pushing updates to applications on events
* Low-latency queue-based or pub-sub messaging
* Fast access to contextual and transactional data via caching patterns such as
read/write-through and write-behind
* Distributed coordination for microservices
* Replicating data from one region to another or between data centers in the
same region
## Key Features
* Stateful and fault-tolerant data processing and querying over data streams
and data at rest using SQL or dataflow API
* A comprehensive library of connectors such as Kafka, Hadoop, S3, RDBMS, JMS
and many more
* Distributed messaging using pub-sub and queues
* Distributed, partitioned, queryable key-value store with event listeners,
which can also be used to store contextual data for enriching event streams
with low latency
* A production-ready Raft-implementation which allows lineralizable (CP)
concurrency primitives such as distributed locks.
* Tight integration for deploying machine learning models with Python to a data
processing pipeline
* Cloud-native, run everywhere architecture
* Zero-downtime operations with rolling upgrades
* At-least-once and exactly-once processing guarantees for stream processing
pipelines
* Data replication between data centers and geographic regions using WAN
* Microsecond performance for key-value point lookups and pub-sub
* Unique data processing architecture results in 99.99% latency of under 10ms
for streaming queries with millions of events per second.
* Client libraries in [Java](https://github.com/hazelcast/hazelcast),
[Python](https://github.com/hazelcast/hazelcast-python-client), [Node.js](https://github.com/hazelcast/hazelcast-nodejs-client), [.NET](https://github.com/hazelcast/hazelcast-csharp-client), [C++](https://github.com/hazelcast/hazelcast-cpp-client) and [Go](https://github.com/hazelcast/hazelcast-go-client)
### Operational Data Store
Hazelcast provides distributed in-memory data structures which are partitioned,
replicated and queryable. One of the main use cases for Hazelcast is for storing
a _working set_ of data for fast querying and access.
The main data structure underlying Hazelcast, called `IMap`, is a key-value store
which has a rich set of features, including:
* Integration with [data
sources](https://docs.hazelcast.com/hazelcast/latest/pipelines/sources-sinks.htm)
for one time or continuous ingestion
* [Read-through and
write-through](https://docs.hazelcast.com/hazelcast/latest/data-structures/map.html#loading-and-storing-persistent-data)
caching patterns
* Indexing and querying through
[SQL](https://docs.hazelcast.com/hazelcast/latest/query/sql-overview.html)
* Processing entries in place for [atomic
updates](https://docs.hazelcast.com/hazelcast/latest/computing/entry-processor.html)
* [Expiring
items](https://docs.hazelcast.com/hazelcast/latest/data-structures/map.html#map-eviction)
automatically based on certain criteria like TTL or last access time
* [Near
cache](https://docs.hazelcast.com/hazelcast/latest/performance/near-cache.html)
for caching entries on the client
* [Listeners](https://docs.hazelcast.com/hazelcast/latest/events/object-events.html)
for pushing changes to clients
* [Data Replication](https://docs.hazelcast.com/hazelcast/latest/wan/wan.html)
between datacenters (Enterprise version only)
* [Persistence](https://docs.hazelcast.com/hazelcast/latest/storage/persistence.html)
of data on disk (Enterprise version only)
Hazelcast stores data in
[partitions](https://docs.hazelcast.com/hazelcast/latest/consistency-and-replication/replication-algorithm.html),
which are distributed to all the nodes. You can increase the storage capacity
by adding additional nodes, and if one of the nodes go down, the data is
restored automatically from the backup replicas.
<img src="images/replication.png"/>
You can interact with maps using SQL or a programming language client of your
choice. You can create and interact with a map as follows:
```sql
CREATE MAPPING myMap (name varchar EXTERNAL NAME "__key", age INT EXTERNAL NAME "this")
TYPE IMap
OPTIONS ('keyFormat'='varchar','valueFormat'='int');
INSERT INTO myMap VALUES('Jake', 29);
SELECT * FROM myMap;
```
The same can be done programmatically as follows using one of the [supported
programming
languages](https://docs.hazelcast.com/hazelcast/latest/clients/hazelcast-clients.html).
Here are some exmaples in Java and Python:
```java
var hz = HazelcastClient.newHazelcastClient();
IMap<String, Integer> map = hz.getMap("myMap");
map.set(Alice, 25);
```
```python
import hazelcast
client = hazelcast.HazelcastClient()
my_map = client.get_map("myMap")
age = my_map.get("Alice").result()
```
Other programming languages supported are
[C#](https://docs.hazelcast.com/hazelcast/latest/clients/dotnet.html),
[C++](https://docs.hazelcast.com/hazelcast/latest/clients/cplusplus.html),
[Node.js](https://docs.hazelcast.com/hazelcast/latest/clients/nodejs.html) and
[Go](https://docs.hazelcast.com/hazelcast/latest/clients/go.html).
Alternatively, you can ingest data directly from the many [sources supported](https://docs.hazelcast.com/hazelcast/latest/pipelines/sources-sinks.html)
using SQL:
```sql
CREATE MAPPING csv_ages (name VARCHAR, age INT)
TYPE File
OPTIONS ('format'='csv',
'path'='/data', 'glob'='data.csv');
SINK INTO myMap
SELECT name, age FROM csv_ages;
```
Hazelcast also provides additional data structures such as ReplicatedMap, Set,
MultiMap and List. For a full list, refer to the [distributed data
structures](https://docs.hazelcast.com/hazelcast/latest/data-structures/distributed-data-structures.html)
section of the docs.
### Stateful Data Processing
Hazelcast has a built-in data processing engine called
[Jet](https://arxiv.org/abs/2103.10169). Jet can be used to build both streaming
and batch data pipelines that are elastic. You can use it to process large
volumes of real-time events or huge batches of static datasets. To give a sense
of scale, a single node of Hazelcast has been proven to [aggregate 10 million
events per second](https://jet-start.sh/blog/2020/08/05/gc-tuning-for-jet) with
latency under 10 milliseconds. A cluster of Hazelcast nodes can process [billion
events per
second](https://hazelcast.com/blog/billion-events-per-second-with-millisecond-latency-streaming-analytics-at-giga-scale/).
<img src="images/latency.png"/>
An application which aggregates millions of sensor readings per
second with 10-millisecond resolution from Kafka looks like the
following:
```java
var hz = Hazelcast.bootstrappedInstance();
var p = Pipeline.create();
p.readFrom(KafkaSources.<String, Reading>kafka(kafkaProperties, "sensors"))
.withTimestamps(event -> event.getValue().timestamp(), 10) // use event timestamp, allowed lag in ms
.groupingKey(reading -> reading.sensorId())
.window(sliding(1_000, 10)) // sliding window of 1s by 10ms
.aggregate(averagingDouble(reading -> reading.temperature()))
.writeTo(Sinks.logger());
hz.getJet().newJob(p).join();
```
Use the following command to deploy the application to the server:
```bash
bin/hazelcast submit analyze-sensors.jar
```
Jet supports advanced streaming features such as [exactly-once processing](https://docs.hazelcast.com/hazelcast/latest/fault-tolerance/fault-tolerance.html) and
[watermarks](https://docs.hazelcast.com/hazelcast/latest/architecture/event-time-processing.html).
#### Data Processing using SQL
Jet also powers the [SQL engine](https://docs.hazelcast.com/hazelcast/latest/query/sql-overview.html)
in Hazelcast which can execute both streaming and batch queries. Internally, all SQL queries
are converted to Jet jobs.
```sql
CREATE MAPPING trades (
id BIGINT,
ticker VARCHAR,
price DECIMAL,
amount BIGINT)
TYPE Kafka
OPTIONS (
'valueFormat' = 'json',
'bootstrap.servers' = 'kafka:9092'
);
SELECT ticker, ROUND(price * 100) AS price_cents, amount
FROM trades
WHERE price * amount > 100;
+------------+----------------------+-------------------+
|ticker | price_cents| amount|
+------------+----------------------+-------------------+
|EFGH | 1400| 20|
```
### Messaging
Hazelcast provides lightweight options for adding messaging to your application.
The two main constructs for messaging are topics and queues.
#### Topics
Topics provide a publish-subscribe pattern where each message is fanned out to
multiple subscribers. See the examples below in Java and Python:
```java
var hz = Hazelcast.bootstrappedInstance();
ITopic<String> topic = hz.getTopic("my_topic");
topic.addMessageListener(msg -> System.out.println(msg));
topic.publish("message");
```
```python
topic = client.get_topic("my_topic")
def handle_message(msg):
print("Received message %s" % msg.message)
topic.add_listener(on_message=handle_message)
topic.publish("my-message")
```
For examples in other languages, please refer to the [docs](https://docs.hazelcast.com/hazelcast/latest/data-structures/topic.html).
#### Queues
Queues provide FIFO-semantics and you can add items from one client and remove
from another. See the examples below in Java and Python:
```java
var client = Hazelcast.newHazelcastClient();
IQueue<String> queue = client.getQueue("my_queue");
queue.put("new-item")
```
```python
import hazelcast
client = hazelcast.HazelcastClient()
q = client.get_queue("my_queue")
my_item = q.take().result()
print("Received item %s" % my_item)
```
For examples in other languages, please refer to the [docs](https://docs.hazelcast.com/hazelcast/latest/data-structures/queue.html).
## Get Started
Follow the [Getting Started
Guide](https://docs.hazelcast.com/hazelcast/latest/getting-started/install-hazelcast)
to install and start using Hazelcast.
## Documentation
Read the [documentation](https://docs.hazelcast.com/) for
in-depth details about how to install Hazelcast and an overview of the features.
## Get Help
You can use [Slack](https://slack.hazelcast.com/) for getting help with Hazelcast
## How to Contribute
Thanks for your interest in contributing! The easiest way is to just send a pull
request. Have a look at the
[issues](https://github.com/hazelcast/hazelcast-jet/issues?q=is%3Aopen+is%3Aissue+label%3A%22good+first+issue%22)
marked as good first issue for some guidance.
### Building From Source
Building Hazelcast requires at minimum JDK 17. Pull the latest source from the
repository and use Maven install (or package) to build:
```bash
$ git pull origin master
$ ./mvnw clean package -DskipTests
```
It is recommended to use the included Maven wrapper script.
It is also possible to use local Maven distribution with the same
version that is used in the Maven wrapper script.
Additionally, there is a `quick` build activated by setting the `-Dquick` system
property that skips validation tasks for faster local builds (e.g. tests, checkstyle
validation, javadoc, source plugins etc) and does not build `extensions` and `distribution`
modules.
### Testing
Take into account that the default build executes thousands of tests which may
take a considerable amount of time. Hazelcast has 3 testing profiles:
* Default:
```bash
./mvnw test
```
to run quick/integration tests (those can be run
in parallel without using network by using `-P parallelTest` profile).
* Slow Tests:
```bash
./mvnw test -P nightly-build
```
to run tests that are either slow
or cannot be run in parallel.
* All Tests:
```bash
./mvnw test -P all-tests
```
to run all tests serially using
network.
Some tests require Docker to run. Set `-Dhazelcast.disable.docker.tests` system property to ignore them.
When developing a PR it is sufficient to run your new tests and some
related subset of tests locally. Our PR builder will take care of running
the full test suite.
## Trigger Phrases in the Pull Request Conversation
When you create a pull request (PR), it must pass a build-and-test
procedure. Maintainers will be notified about your PR, and they can
trigger the build using special comments. These are the phrases you may
see used in the comments on your PR:
* `run-lab-run` - run the default PR builder
* `run-lts-compilers` - compiles the sources with JDK 17 and JDK 21 (without running tests)
* `run-ee-compile` - compile hazelcast-enterprise with this PR
* `run-ee-tests` - run tests from hazelcast-enterprise with this PR
* `run-windows` - run the tests on a Windows machine (HighFive is not supported here)
* `run-with-ibm-jdk-8` - run the tests with IBM JDK 8
* `run-cdc-debezium-tests` - run all tests in the
`extensions/cdc-debezium` module
* `run-cdc-mysql-tests` - run all tests in the `extensions/cdc-mysql`
module
* `run-cdc-postgres-tests` - run all tests in the
`extensions/cdc-postgres` module
* `run-mongodb-tests` - run all tests in the `extensions/mongodb` module
* `run-s3-tests` - run all tests in the `extensions/s3` module
* *`run-nightly-tests` - run nightly (slow) tests. WARNING: Use with care as this is a resource consuming task.*
* *`run-ee-nightly-tests` - run nightly (slow) tests from hazelcast-enterprise. WARNING: Use with care as this is a resource consuming task.*
* `run-sql-only` - run default tests in `hazelcast-sql`, `hazelcast-distribution`, and `extensions/mapstore` modules
* `run-docs-only` - do not run any tests, check that only files with `.md`, `.adoc` or `.txt` suffix are added in the PR
* `run-sonar` - run SonarCloud analysis
* `run-arm64` - run the tests on arm64 machine
Where not indicated, the builds run on a Linux machine with Oracle JDK 17.
### Creating PRs for Hazelcast SQL
When creating a PR with changes located in the `hazelcast-sql` module and nowhere else,
you can label your PR with `SQL-only`. This will change the standard PR builder to one that
will only run tests related to SQL (see `run-sql-only` above), which will significantly shorten
the build time vs. the default PR builder. **NOTE**: this job will fail if you've made changes
anywhere other than `hazelcast-sql`.
### Creating PRs which contain only documentation
When creating a PR which changes only documentation (files with suffix `.md` or `.adoc`) it
makes no sense to run tests. For that case the label `docs-only` can be used. The job will fail
in case you've made other changes than in `.md`, `.adoc` or `.txt` files.
## License
Source code in this repository is covered by one of two licenses:
1. [Apache License 2.0](https://docs.hazelcast.com/hazelcast/latest/index.html#licenses-and-support)
2. [Hazelcast Community
License](http://hazelcast.com/hazelcast-community-license)
The default license throughout the repository is Apache License 2.0 unless the
header specifies another license.
## Acknowledgments
[](http://www.yourkit.com/)
Thanks to [YourKit](http://www.yourkit.com/) for supporting open source software
by providing us a free license for their Java profiler.
We owe (the good parts of) our CLI tool's user experience to
[picocli](https://picocli.info/).
## Copyright
Copyright (c) 2008-2024, Hazelcast, Inc. All Rights Reserved.
Visit [www.hazelcast.com](http://www.hazelcast.com/) for more info.
| 0 |
xuhuisheng/lemon | 开源OA | null | lemon
=====
[](https://travis-ci.org/xuhuisheng/lemon)
开源OA
http://www.mossle.com/
| 0 |
portfolio-performance/portfolio | Track and evaluate the performance of your investment portfolio across stocks, cryptocurrencies, and other assets. | eclipse financial investment-portfolio java portfolio portfolio-performance stocks | # About
[Portfolio Performance](https://www.portfolio-performance.info): Track and evaluate the performance of your investment portfolio across stocks, cryptocurrencies, and other assets.
## Status
[](https://github.com/portfolio-performance/portfolio/actions?query=workflow%3ACI) [](https://github.com/portfolio-performance/portfolio/releases/latest) [](https://github.com/portfolio-performance/portfolio/releases/latest) [](https://github.com/portfolio-performance/portfolio/blob/master/LICENSE)
[](https://sonarcloud.io/dashboard?id=name.abuchen.portfolio%3Aportfolio-app) [](https://sonarcloud.io/project/issues?id=name.abuchen.portfolio%3Aportfolio-app&resolved=false&types=BUG) [](https://sonarcloud.io/project/issues?id=name.abuchen.portfolio%3Aportfolio-app&resolved=false&types=VULNERABILITY) [](https://sonarcloud.io/component_measures?id=name.abuchen.portfolio%3Aportfolio-app&metric=Coverage)
## Links
* [Homepage](https://www.portfolio-performance.info)
* [Downloads](https://github.com/portfolio-performance/portfolio/releases)
* [Forum](https://forum.portfolio-performance.info/)
* [Help (German)](https://help.portfolio-performance.info/)
## Contributing Source Code
* [Development setup](CONTRIBUTING.md#development-setup)
* [Project setup](CONTRIBUTING.md#project-setup)
* [Contribute code](CONTRIBUTING.md#contribute-code)
* [Images, Logo & Colors](CONTRIBUTING.md#images-logo-and-color)
* [Translations](CONTRIBUTING.md#translations)
* [Interactive-Flex-Query importer](CONTRIBUTING.md#interactive-flex-query-importer)
* [PDF importer](CONTRIBUTING.md#pdf-importer)
* [Trade calendar](CONTRIBUTING.md#trade-calendar)
## License
Eclipse Public License
https://www.eclipse.org/legal/epl-v10.html
| 0 |