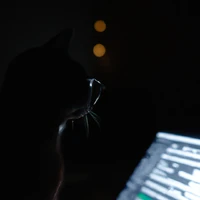
rjmacarthy/codegen-350M-finetuned-react
Text Generation
•
Updated
•
22
•
1
path
stringlengths 5
195
| repo_name
stringlengths 5
79
| content
stringlengths 25
1.01M
|
---|---|---|
docs/app/Examples/modules/Dropdown/Usage/DropdownExampleCustomNoResultsMessage.js | mohammed88/Semantic-UI-React | import React from 'react'
import { Dropdown } from 'semantic-ui-react'
const DropdownExampleCustomNoResultsMessage = () => (
<Dropdown
options={[]}
search
selection
placeholder='A custom message...'
noResultsMessage='Try another search.'
/>
)
export default DropdownExampleCustomNoResultsMessage
|
src/components/animated_box.js | rdenadai/mockingbird | import { css } from '../css';
import React, { Component } from 'react';
import { findDOMNode } from 'react-dom';
import Velocity from 'velocity-animate';
// const uuid = require('uuid');
class AnimatedBox extends Component {
constructor(props) {
super(props);
this.state = { mounted: false };
}
componentWillMount() {
// silence
}
componentWillAppear(callback) {
// const el = findDOMNode(this);
const el = findDOMNode(this);
Velocity(el, { opacity: 1 }, { visibility: 'visible' }, 800)
.then(() => {
this.setState({ mounted: true });
callback();
});
}
componentWillEnter(callback) {
// const el = findDOMNode(this);
callback();
}
componentDidEnter() {
const el = findDOMNode(this);
Velocity(el, { opacity: 1 }, { visibility: 'visible' }, 800)
.then(() => {
this.setState({ mounted: true });
});
}
componentWillLeave(callback) {
const el = findDOMNode(this);
Velocity(el, { opacity: 0 }, { visibility: 'hidden' }, { delay: 250, duration: 800 })
.then(() => {
this.setState({ mounted: false });
callback();
});
}
render() {
const children = !!this.props.children ? this.props.children : null;
return (
<div className={css.baseCSS.animatedBox + ' ' + css.baseCSS.fullHeight}>
{children}
</div>
);
}
}
AnimatedBox.propTypes = {
id: React.PropTypes.string,
children: React.PropTypes.node
};
export default AnimatedBox;
|
app/javascript/flavours/glitch/features/direct_timeline/components/conversation.js | glitch-soc/mastodon | import React from 'react';
import PropTypes from 'prop-types';
import ImmutablePropTypes from 'react-immutable-proptypes';
import ImmutablePureComponent from 'react-immutable-pure-component';
import StatusContent from 'flavours/glitch/components/status_content';
import AttachmentList from 'flavours/glitch/components/attachment_list';
import { defineMessages, injectIntl, FormattedMessage } from 'react-intl';
import DropdownMenuContainer from 'flavours/glitch/containers/dropdown_menu_container';
import AvatarComposite from 'flavours/glitch/components/avatar_composite';
import Permalink from 'flavours/glitch/components/permalink';
import IconButton from 'flavours/glitch/components/icon_button';
import RelativeTimestamp from 'flavours/glitch/components/relative_timestamp';
import { HotKeys } from 'react-hotkeys';
import { autoPlayGif } from 'flavours/glitch/util/initial_state';
import classNames from 'classnames';
const messages = defineMessages({
more: { id: 'status.more', defaultMessage: 'More' },
open: { id: 'conversation.open', defaultMessage: 'View conversation' },
reply: { id: 'status.reply', defaultMessage: 'Reply' },
markAsRead: { id: 'conversation.mark_as_read', defaultMessage: 'Mark as read' },
delete: { id: 'conversation.delete', defaultMessage: 'Delete conversation' },
muteConversation: { id: 'status.mute_conversation', defaultMessage: 'Mute conversation' },
unmuteConversation: { id: 'status.unmute_conversation', defaultMessage: 'Unmute conversation' },
});
export default @injectIntl
class Conversation extends ImmutablePureComponent {
static contextTypes = {
router: PropTypes.object,
};
static propTypes = {
conversationId: PropTypes.string.isRequired,
accounts: ImmutablePropTypes.list.isRequired,
lastStatus: ImmutablePropTypes.map,
unread:PropTypes.bool.isRequired,
scrollKey: PropTypes.string,
onMoveUp: PropTypes.func,
onMoveDown: PropTypes.func,
markRead: PropTypes.func.isRequired,
delete: PropTypes.func.isRequired,
intl: PropTypes.object.isRequired,
};
state = {
isExpanded: undefined,
};
parseClick = (e, destination) => {
const { router } = this.context;
const { lastStatus, unread, markRead } = this.props;
if (!router) return;
if (e.button === 0 && !(e.ctrlKey || e.altKey || e.metaKey)) {
if (destination === undefined) {
if (unread) {
markRead();
}
destination = `/statuses/${lastStatus.get('id')}`;
}
let state = {...router.history.location.state};
state.mastodonBackSteps = (state.mastodonBackSteps || 0) + 1;
router.history.push(destination, state);
e.preventDefault();
}
}
handleMouseEnter = ({ currentTarget }) => {
if (autoPlayGif) {
return;
}
const emojis = currentTarget.querySelectorAll('.custom-emoji');
for (var i = 0; i < emojis.length; i++) {
let emoji = emojis[i];
emoji.src = emoji.getAttribute('data-original');
}
}
handleMouseLeave = ({ currentTarget }) => {
if (autoPlayGif) {
return;
}
const emojis = currentTarget.querySelectorAll('.custom-emoji');
for (var i = 0; i < emojis.length; i++) {
let emoji = emojis[i];
emoji.src = emoji.getAttribute('data-static');
}
}
handleClick = () => {
if (!this.context.router) {
return;
}
const { lastStatus, unread, markRead } = this.props;
if (unread) {
markRead();
}
this.context.router.history.push(`/@${lastStatus.getIn(['account', 'acct'])}/${lastStatus.get('id')}`);
}
handleMarkAsRead = () => {
this.props.markRead();
}
handleReply = () => {
this.props.reply(this.props.lastStatus, this.context.router.history);
}
handleDelete = () => {
this.props.delete();
}
handleHotkeyMoveUp = () => {
this.props.onMoveUp(this.props.conversationId);
}
handleHotkeyMoveDown = () => {
this.props.onMoveDown(this.props.conversationId);
}
handleConversationMute = () => {
this.props.onMute(this.props.lastStatus);
}
handleShowMore = () => {
if (this.props.lastStatus.get('spoiler_text')) {
this.setExpansion(!this.state.isExpanded);
}
};
setExpansion = value => {
this.setState({ isExpanded: value });
}
render () {
const { accounts, lastStatus, unread, scrollKey, intl } = this.props;
const { isExpanded } = this.state;
if (lastStatus === null) {
return null;
}
const menu = [
{ text: intl.formatMessage(messages.open), action: this.handleClick },
null,
];
menu.push({ text: intl.formatMessage(lastStatus.get('muted') ? messages.unmuteConversation : messages.muteConversation), action: this.handleConversationMute });
if (unread) {
menu.push({ text: intl.formatMessage(messages.markAsRead), action: this.handleMarkAsRead });
menu.push(null);
}
menu.push({ text: intl.formatMessage(messages.delete), action: this.handleDelete });
const names = accounts.map(a => <Permalink to={`/@${a.get('acct')}`} href={a.get('url')} key={a.get('id')} title={a.get('acct')}><bdi><strong className='display-name__html' dangerouslySetInnerHTML={{ __html: a.get('display_name_html') }} /></bdi></Permalink>).reduce((prev, cur) => [prev, ', ', cur]);
const handlers = {
reply: this.handleReply,
open: this.handleClick,
moveUp: this.handleHotkeyMoveUp,
moveDown: this.handleHotkeyMoveDown,
toggleHidden: this.handleShowMore,
};
let media = null;
if (lastStatus.get('media_attachments').size > 0) {
media = <AttachmentList compact media={lastStatus.get('media_attachments')} />;
}
return (
<HotKeys handlers={handlers}>
<div className={classNames('conversation focusable muted', { 'conversation--unread': unread })} tabIndex='0'>
<div className='conversation__avatar' onClick={this.handleClick} role='presentation'>
<AvatarComposite accounts={accounts} size={48} />
</div>
<div className='conversation__content'>
<div className='conversation__content__info'>
<div className='conversation__content__relative-time'>
{unread && <span className='conversation__unread' />} <RelativeTimestamp timestamp={lastStatus.get('created_at')} />
</div>
<div className='conversation__content__names' onMouseEnter={this.handleMouseEnter} onMouseLeave={this.handleMouseLeave}>
<FormattedMessage id='conversation.with' defaultMessage='With {names}' values={{ names: <span>{names}</span> }} />
</div>
</div>
<StatusContent
status={lastStatus}
parseClick={this.parseClick}
expanded={isExpanded}
onExpandedToggle={this.handleShowMore}
collapsable
media={media}
/>
<div className='status__action-bar'>
<IconButton className='status__action-bar-button' title={intl.formatMessage(messages.reply)} icon='reply' onClick={this.handleReply} />
<div className='status__action-bar-dropdown'>
<DropdownMenuContainer
scrollKey={scrollKey}
status={lastStatus}
items={menu}
icon='ellipsis-h'
size={18}
direction='right'
title={intl.formatMessage(messages.more)}
/>
</div>
</div>
</div>
</div>
</HotKeys>
);
}
}
|
frontend/src/DiscoverMovie/Exclusion/ExcludeMovieModal.js | geogolem/Radarr | import PropTypes from 'prop-types';
import React from 'react';
import Modal from 'Components/Modal/Modal';
import { sizes } from 'Helpers/Props';
import ExcludeMovieModalContentConnector from './ExcludeMovieModalContentConnector';
function ExcludeMovieModal(props) {
const {
isOpen,
onModalClose,
...otherProps
} = props;
return (
<Modal
isOpen={isOpen}
size={sizes.MEDIUM}
onModalClose={onModalClose}
>
<ExcludeMovieModalContentConnector
{...otherProps}
onModalClose={onModalClose}
/>
</Modal>
);
}
ExcludeMovieModal.propTypes = {
isOpen: PropTypes.bool.isRequired,
onModalClose: PropTypes.func.isRequired
};
export default ExcludeMovieModal;
|
django/webcode/webcode/frontend/node_modules/react-router-dom/es/Link.js | OpenKGB/webcode | var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
function _objectWithoutProperties(obj, keys) { var target = {}; for (var i in obj) { if (keys.indexOf(i) >= 0) continue; if (!Object.prototype.hasOwnProperty.call(obj, i)) continue; target[i] = obj[i]; } return target; }
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
import React from 'react';
import PropTypes from 'prop-types';
var isModifiedEvent = function isModifiedEvent(event) {
return !!(event.metaKey || event.altKey || event.ctrlKey || event.shiftKey);
};
/**
* The public API for rendering a history-aware <a>.
*/
var Link = function (_React$Component) {
_inherits(Link, _React$Component);
function Link() {
var _temp, _this, _ret;
_classCallCheck(this, Link);
for (var _len = arguments.length, args = Array(_len), _key = 0; _key < _len; _key++) {
args[_key] = arguments[_key];
}
return _ret = (_temp = (_this = _possibleConstructorReturn(this, _React$Component.call.apply(_React$Component, [this].concat(args))), _this), _this.handleClick = function (event) {
if (_this.props.onClick) _this.props.onClick(event);
if (!event.defaultPrevented && // onClick prevented default
event.button === 0 && // ignore right clicks
!_this.props.target && // let browser handle "target=_blank" etc.
!isModifiedEvent(event) // ignore clicks with modifier keys
) {
event.preventDefault();
var history = _this.context.router.history;
var _this$props = _this.props,
replace = _this$props.replace,
to = _this$props.to;
if (replace) {
history.replace(to);
} else {
history.push(to);
}
}
}, _temp), _possibleConstructorReturn(_this, _ret);
}
Link.prototype.render = function render() {
var _props = this.props,
replace = _props.replace,
to = _props.to,
props = _objectWithoutProperties(_props, ['replace', 'to']); // eslint-disable-line no-unused-vars
var href = this.context.router.history.createHref(typeof to === 'string' ? { pathname: to } : to);
return React.createElement('a', _extends({}, props, { onClick: this.handleClick, href: href }));
};
return Link;
}(React.Component);
Link.propTypes = {
onClick: PropTypes.func,
target: PropTypes.string,
replace: PropTypes.bool,
to: PropTypes.oneOfType([PropTypes.string, PropTypes.object]).isRequired
};
Link.defaultProps = {
replace: false
};
Link.contextTypes = {
router: PropTypes.shape({
history: PropTypes.shape({
push: PropTypes.func.isRequired,
replace: PropTypes.func.isRequired,
createHref: PropTypes.func.isRequired
}).isRequired
}).isRequired
};
export default Link; |
example/src/components/0-Logo/Logo.js | KenPowers/css-modules-electron | import styles from './Logo.css';
import React, { Component } from 'react';
export default class Logo extends Component {
render() {
return <div className={styles.logo} />;
}
};
|
src/containers/_base/stories/index.js | timludikar/component-library | import React from 'react';
import { storiesOf } from '@kadira/storybook';
import BaseContainer from '../index';
storiesOf('container.BaseContainer', module)
.add('default view', () => {
return (
<BaseContainer title="Base Container">TEST</BaseContainer>
);
});
|
src/parser/hunter/beastmastery/modules/talents/ChimaeraShot.js | fyruna/WoWAnalyzer | import React from 'react';
import Analyzer from 'parser/core/Analyzer';
import SPELLS from 'common/SPELLS';
import SpellLink from 'common/SpellLink';
import ItemDamageDone from 'interface/others/ItemDamageDone';
import StatisticListBoxItem from 'interface/others/StatisticListBoxItem';
import TalentStatisticBox from 'interface/others/TalentStatisticBox';
import AverageTargetsHit from 'interface/others/AverageTargetsHit';
/**
* A two-headed shot that hits your primary target and another nearby target, dealing 720% Nature damage to one and 720% Frost damage to the other.
*
* Example log: https://www.warcraftlogs.com/reports/PLyFT2hcmCv39X7R#fight=1&type=damage-done
*/
class ChimaeraShot extends Analyzer {
damage = 0;
casts = 0;
hits = 0;
constructor(...args) {
super(...args);
this.active = this.selectedCombatant.hasTalent(SPELLS.CHIMAERA_SHOT_TALENT.id);
}
on_byPlayer_cast(event) {
const spellId = event.ability.guid;
if (spellId !== SPELLS.CHIMAERA_SHOT_TALENT.id) {
return;
}
this.casts += 1;
}
on_byPlayer_damage(event) {
const spellId = event.ability.guid;
if (spellId !== SPELLS.CHIMAERA_SHOT_FROST_DAMAGE.id && spellId !== SPELLS.CHIMAERA_SHOT_NATURE_DAMAGE.id) {
return;
}
this.hits += 1;
this.damage += event.amount + (event.absorbed || 0);
}
statistic() {
return (
<TalentStatisticBox
talent={SPELLS.CHIMAERA_SHOT_TALENT.id}
value={<>
<AverageTargetsHit casts={this.casts} hits={this.hits} />
</>}
/>
);
}
subStatistic() {
return (
<StatisticListBoxItem
title={<SpellLink id={SPELLS.CHIMAERA_SHOT_TALENT.id} />}
value={<ItemDamageDone amount={this.damage} />}
/>
);
}
}
export default ChimaeraShot;
|
src/scripts/base/Strong.js | ButuzGOL/constructor | import React from 'react';
export default class Strong extends React.Component {
getStyles() {
return {
fontWeight: 'bold'
};
}
render() {
return (
<strong style={this.getStyles()}>
{this.props.children}
</strong>
);
}
}
|
js/components/inputgroup/regular.js | ChiragHindocha/stay_fit |
import React, { Component } from 'react';
import { connect } from 'react-redux';
import { actions } from 'react-native-navigation-redux-helpers';
import { Container, Header, Title, Content, Button, Icon, Text, Body, Left, Right, Input, Item } from 'native-base';
import { Actions } from 'react-native-router-flux';
import styles from './styles';
const {
popRoute,
} = actions;
class Regular extends Component {
static propTypes = {
popRoute: React.PropTypes.func,
navigation: React.PropTypes.shape({
key: React.PropTypes.string,
}),
}
popRoute() {
this.props.popRoute(this.props.navigation.key);
}
render() {
return (
<Container style={styles.container}>
<Header>
<Left>
<Button transparent onPress={() => Actions.pop()}>
<Icon name="arrow-back" />
</Button>
</Left>
<Body>
<Title>Regular</Title>
</Body>
<Right />
</Header>
<Content padder>
<Item regular>
<Input placeholder="Regular Textbox" />
</Item>
</Content>
</Container>
);
}
}
function bindAction(dispatch) {
return {
popRoute: key => dispatch(popRoute(key)),
};
}
const mapStateToProps = state => ({
navigation: state.cardNavigation,
themeState: state.drawer.themeState,
});
export default connect(mapStateToProps, bindAction)(Regular);
|
server/priv/js/components/BenchNav.react.js | bks7/mzbench | import React from 'react';
class BenchNav extends React.Component {
render() {
const tabs = {
overview: "Overview",
scenario: "Scenario",
reports: "Reports",
logs: "Logs"
};
return (
<ul className="nav nav-tabs bench-nav">
{Object.keys(tabs).map(function (tab) {
let name = tabs[tab];
let cssClass = (this.props.selectedTab == tab) ? "active" : "";
let link = `#/bench/${this.props.bench.id}/${tab}`;
return (<li role="presentation" key={tab} className={cssClass}><a href={link}>{name}</a></li>);
}.bind(this))}
</ul>
);
}
};
BenchNav.propTypes = {
bench: React.PropTypes.object.isRequired,
selectedTab: React.PropTypes.string
};
BenchNav.defaultProps = {
selectedTab: "overview"
};
export default BenchNav;
|
dev/component/multiSelectionGroup.js | TedForV/EFS | import React from 'react';
import RSelect from 'react-select';
import Immutable from 'immutable';
import gStyle from '../globalStyle';
require('../../node_modules/react-select/dist/react-select.min.css');
class MultiSelect extends React.Component {
constructor(props) {
super(props);
this.filterName = this.props.filterName
this.state = {
options : this.orderOption(this.props.options),
values : this.props.value
}
this._onSelectChange = this._onSelectChange.bind(this);
this.onSelectChange = this.props.onSelectChange;
}
orderOption(options) {
return options.sort((a, b) => {
if (a.label > b.label) {
return 1;
} else if (a.label == b.label) {
return 0;
} else {
return -1;
}
});
}
_onSelectChange(value){
this.setState({
values:value
});
this.onSelectChange({
filterName:this.filterName,
selectValues:value
});
}
componentWillReceiveProps(nextProps){
this.setState({
options : this.orderOption(nextProps.options)
});
}
render() {
var placeholder = 'Input ' + this.filterName;
return (
<div className="col-md-12 margin-botton-div">
<RSelect multi simpleValue delimiter={gStyle.constV.delimiter} value={this.state.values} placeholder={placeholder} options={this.state.options} onChange={this._onSelectChange} />
</div>
);
}
}
class MultiSelectGroup extends React.Component {
constructor(props) {
super(props);
this.state={
filterOptions:Immutable.Map(this.props.filterOptions)
};
this._initialFilter(this.props.currentFilter)
this._onSelectChange = this._onSelectChange.bind(this);
}
_initialFilter(currentFilter){
this.filter = Immutable.Map({});
for(var key of this.state.filterOptions.keys()){
this.filter.set(key,'');
}
if(currentFilter){
var currFilter =Immutable.Map(currentFilter);
this.filter = this.filter.merge(currFilter);
}
}
_onSelectChange(selectValue){
this.props.onFilterChange(selectValue);
}
componentWillReceiveProps(nextProps){
this.setState({
filterOptions:Immutable.Map(nextProps.filterOptions)
});
this._initialFilter(nextProps.currentFilter);
}
render() {
var {filterOptions} = this.state;
var mutilSelects=[];
for(var [key,value] of this.state.filterOptions.entries()){
mutilSelects.push(<MultiSelect key={key} value={this.filter.get(key)} filterName={key} options={value.toArray()} onSelectChange={this._onSelectChange} />);
}
return(
<div className="row">
{mutilSelects}
</div>
);
}
}
module.exports = MultiSelectGroup;
|
src/icons/SearchIcon.js | kiloe/ui | import React from 'react';
import Icon from '../Icon';
export default class SearchIcon extends Icon {
getSVG(){return <svg xmlns="http://www.w3.org/2000/svg" width="48" height="48" viewBox="0 0 48 48"><path d="M31 28h-1.59l-.55-.55C30.82 25.18 32 22.23 32 19c0-7.18-5.82-13-13-13S6 11.82 6 19s5.82 13 13 13c3.23 0 6.18-1.18 8.45-3.13l.55.55V31l10 9.98L40.98 38 31 28zm-12 0c-4.97 0-9-4.03-9-9s4.03-9 9-9 9 4.03 9 9-4.03 9-9 9z"/></svg>;}
}; |
src/scenes/home/landing/galaBanner/galaBanner.js | miaket/operationcode_frontend | import React from 'react';
import { Link } from 'react-router-dom';
import styles from './galaBanner.css';
const GalaBanner = () => (
<div className={styles.galaBannerWrapper}>
<Link to="/gala">
<img className={styles.galaImage} alt="gala banner" />
</Link>
</div>
);
export default GalaBanner;
|
docs/app/Examples/elements/Segment/Groups/SegmentExampleRaisedSegments.js | Rohanhacker/Semantic-UI-React | import React from 'react'
import { Segment } from 'semantic-ui-react'
const SegmentExampleRaisedSegments = () => (
<Segment.Group raised>
<Segment>Left</Segment>
<Segment>Middle</Segment>
<Segment>Right</Segment>
</Segment.Group>
)
export default SegmentExampleRaisedSegments
|
shared/components/error-boundary/ErrorBoundary.js | ueno-llc/starter-kit-universally | import React, { Component } from 'react';
import PropTypes from 'prop-types';
export default class ErrorBoundary extends Component {
static propTypes = {
children: PropTypes.node,
}
state = {
error: null,
errorInfo: null,
};
componentDidCatch(error, errorInfo) {
this.setState({
error,
errorInfo,
});
}
render() {
if (this.state.errorInfo) {
return (
<div>
<h2>Error</h2>
<p>{this.state.error && this.state.error.toString()}</p>
<pre>{this.state.errorInfo.componentStack}</pre>
</div>
);
}
return this.props.children;
}
}
|
src/parser/hunter/beastmastery/CHANGELOG.js | fyruna/WoWAnalyzer | import React from 'react';
import { Putro, Streammz } from 'CONTRIBUTORS';
import SpellLink from 'common/SpellLink';
import SPELLS from 'common/SPELLS';
export default [
{
date: new Date('2018-11-14'),
changes: <> Created a module for <SpellLink id={SPELLS.BORN_TO_BE_WILD_TALENT.id} /> and <SpellLink id={SPELLS.BINDING_SHOT_TALENT.id} />. </>,
contributors: [Putro],
},
{
date: new Date('2018-11-13'),
changes: <> Implemented talent statistic module for <SpellLink id={SPELLS.ANIMAL_COMPANION_TALENT.id} /> & an azerite power module for Pack Alpha. </>,
contributors: [Putro],
},
{
date: new Date('2018-11-13'),
changes: <> Implemented talent statistic modules for <SpellLink id={SPELLS.BARRAGE_TALENT.id} /> and <SpellLink id={SPELLS.CHIMAERA_SHOT_TALENT.id} />.</>,
contributors: [Putro],
},
{
date: new Date('2018-11-07'),
changes: <>Implemented talent statistic modules for <SpellLink id={SPELLS.ONE_WITH_THE_PACK_TALENT.id} /> and <SpellLink id={SPELLS.STOMP_TALENT.id} />.</>,
contributors: [Putro],
},
{
date: new Date('2018-11-08'),
changes: <> Implemented a module for <SpellLink id={SPELLS.ASPECT_OF_THE_BEAST_TALENT.id} />, <SpellLink id={SPELLS.SPITTING_COBRA_TALENT.id} />, <SpellLink id={SPELLS.SCENT_OF_BLOOD_TALENT.id} /> and updated the module for <SpellLink id={SPELLS.DIRE_BEAST_TALENT.id} />.</>,
contributors: [Putro],
},
{
date: new Date('2018-11-02'),
changes: <>Implemented a talent statistic module and suggestions for <SpellLink id={SPELLS.VENOMOUS_BITE_TALENT.id} /> and <SpellLink id={SPELLS.THRILL_OF_THE_HUNT_TALENT.id} />.</>,
contributors: [Putro],
},
{
date: new Date('2018-10-31'),
changes: <>Implemented statistics for the talent <SpellLink id={SPELLS.KILLER_INSTINCT_TALENT.id} />.</>,
contributors: [Streammz],
},
{
date: new Date('2018-10-29'),
changes: <>Implemented a suggestion that checks for <SpellLink id={SPELLS.MULTISHOT_BM.id} /> usage in single target situations, warning you about multi-shot uses where no paired <SpellLink id={SPELLS.BEAST_CLEAVE_PET_BUFF.id} /> damage has been dealt.</>,
contributors: [Streammz],
},
{
date: new Date('2018-10-25'),
changes: <>Implemented the new checklist for Beast Mastery, added a <SpellLink id={SPELLS.COBRA_SHOT.id} /> statistic and associated suggestions, and updated <SpellLink id={SPELLS.KILLER_COBRA_TALENT.id} /> suggestions.</>,
contributors: [Putro],
},
{
date: new Date('2018-10-24'),
changes: <>Merged the 3 <SpellLink id={SPELLS.BESTIAL_WRATH.id} /> modules together, and added a cooldown reduction efficiency metric and suggestion to it.</>,
contributors: [Putro],
},
{
date: new Date('2018-09-20'),
changes: <>Added two azerite trait modules, one for <SpellLink id={SPELLS.PRIMAL_INSTINCTS.id} /> and an initial version for <SpellLink id={SPELLS.FEEDING_FRENZY.id} /></>,
contributors: [Putro],
},
{
date: new Date('2018-08-12'),
changes: <>Updated the <SpellLink id={SPELLS.BARBED_SHOT.id} /> statistic to be an expandable statistic box, to showcase uptime of 0->3 stacks separately.</>,
contributors: [Putro],
},
{
date: new Date('2018-08-12'),
changes: 'Removed all legendaries and tier gear in preparation for Battle for Azeroth launch',
contributors: [Putro],
},
{
date: new Date('2018-08-06'),
changes: <> Adds buff indicators to relevant spells in the timeline, adjusted placement of statistic boxes, added example logs to everything BFA related and added statistics for <SpellLink id={SPELLS.CHIMAERA_SHOT_TALENT.id} />, <SpellLink id={SPELLS.DIRE_BEAST_TALENT.id} />, <SpellLink id={SPELLS.KILLER_COBRA_TALENT.id} />, <SpellLink id={SPELLS.STAMPEDE_TALENT.id} /> and <SpellLink id={SPELLS.STOMP_TALENT.id} />. </>,
contributors: [Putro],
},
{
date: new Date('2018-07-26'),
changes: <>Updated the BM module to 8.0.1, and added a <SpellLink id={SPELLS.BARBED_SHOT.id} /> statistic and acoompanying suggestions, also updated downtime module to be accurate with Aspect of the Wilds reduced GCD. </>,
contributors: [Putro],
},
];
|
src/modules/Synth/components/OscillatorBank/index.js | ruebel/synth-react-redux | import React from 'react';
import PropTypes from 'prop-types';
import { connect } from 'react-redux';
import styled from 'styled-components';
import Container from '../../../components/Container';
import Oscillator from './Oscillator';
import RoundButton from '../../../components/Button/RoundButton';
import * as actions from '../../actions';
import { getOscillators } from '../../selectors';
const Bank = styled.div`
display: flex;
flex-direction: row;
align-items: flex-start;
& > div:not(:first-of-type) {
margin-left: 5px;
}
`;
const OscillatorBank = ({
addOscillator,
oscillators,
setOscillatorSetting,
removeOscillator
}) => {
const addButton = <RoundButton active click={addOscillator} text="+" />;
return (
<Container full title="Oscillators" titleControl={addButton}>
<Bank>
{oscillators.map((o, i) => {
return (
<Oscillator
key={i}
oscillator={o}
remove={removeOscillator}
setValue={setOscillatorSetting}
/>
);
})}
</Bank>
</Container>
);
};
OscillatorBank.propTypes = {
addOscillator: PropTypes.func.isRequired,
oscillators: PropTypes.array.isRequired,
setOscillatorSetting: PropTypes.func.isRequired,
removeOscillator: PropTypes.func.isRequired
};
const mapStateToProps = state => {
return {
oscillators: getOscillators(state)
};
};
export default connect(mapStateToProps, actions)(OscillatorBank);
|
src/containers/ui/Menu/MenuView.js | arayi/SLions | /**
* Menu Contents
*
* React Native Starter App
* https://github.com/mcnamee/react-native-starter-app
*/
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import {
View,
Alert,
StyleSheet,
TouchableOpacity,
} from 'react-native';
import { Actions } from 'react-native-router-flux';
// Consts and Libs
import { AppStyles, AppSizes } from '@theme/';
// Components
import { Spacer, Text, Button } from '@ui/';
/* Styles ==================================================================== */
const MENU_BG_COLOR = '#263137';
const styles = StyleSheet.create({
backgroundFill: {
backgroundColor: MENU_BG_COLOR,
height: AppSizes.screen.height,
width: AppSizes.screen.width,
position: 'absolute',
top: 0,
left: 0,
},
container: {
position: 'relative',
flex: 1,
},
menuContainer: {
flex: 1,
left: 0,
right: 0,
backgroundColor: MENU_BG_COLOR,
},
// Main Menu
menu: {
flex: 3,
left: 0,
right: 0,
backgroundColor: MENU_BG_COLOR,
padding: 20,
paddingTop: AppSizes.statusBarHeight + 20,
},
menuItem: {
borderBottomWidth: 1,
borderBottomColor: 'rgba(255, 255, 255, 0.1)',
paddingBottom: 10,
},
menuItem_text: {
fontSize: 16,
lineHeight: parseInt(16 + (16 * 0.5), 10),
fontWeight: '500',
marginTop: 14,
marginBottom: 8,
color: '#EEEFF0',
},
// Menu Bottom
menuBottom: {
flex: 1,
left: 0,
right: 0,
justifyContent: 'flex-end',
paddingBottom: 10,
},
menuBottom_text: {
color: '#EEEFF0',
},
});
/* Component ==================================================================== */
class Menu extends Component {
static propTypes = {
logout: PropTypes.func.isRequired,
closeSideMenu: PropTypes.func.isRequired,
user: PropTypes.shape({
email: PropTypes.string,
}),
unauthMenu: PropTypes.arrayOf(PropTypes.shape({})),
authMenu: PropTypes.arrayOf(PropTypes.shape({})),
}
static defaultProps = {
user: null,
unauthMenu: [],
authMenu: [],
}
/**
* On Press of any menu item
*/
onPress = (action) => {
this.props.closeSideMenu();
if (action) action();
}
/**
* On Logout Press
*/
logout = () => {
if (this.props.logout) {
this.props.logout()
.then(() => {
this.props.closeSideMenu();
Actions.login();
}).catch(() => {
Alert.alert('Oh uh!', 'Something went wrong.');
});
}
}
/**
* Each Menu Item looks like this
*/
menuItem = item => (
<TouchableOpacity
key={`menu-item-${item.title}`}
onPress={() => this.onPress(item.onPress)}
>
<View style={[styles.menuItem]}>
<Text style={[styles.menuItem_text]}>
{item.title}
</Text>
</View>
</TouchableOpacity>
)
/**
* Build the Menu List
*/
menuList = () => {
// Determine which menu to use - authenticated user menu or unauthenicated version?
let menu = this.props.unauthMenu;
if (this.props.user && this.props.user.email) menu = this.props.authMenu;
return menu.map(item => this.menuItem(item));
}
render = () => (
<View style={[styles.container]}>
<View style={[styles.backgroundFill]} />
<View style={[styles.menuContainer]}>
<View style={[styles.menu]}>{this.menuList()}</View>
<View style={[styles.menuBottom]}>
{this.props.user && this.props.user.email ?
<View>
<Text
style={[
styles.menuBottom_text,
AppStyles.textCenterAligned,
]}
>
Logged in as:{'\n'}
{this.props.user.email}
</Text>
<Spacer size={10} />
<View style={[AppStyles.paddingHorizontal, AppStyles.paddingVerticalSml]}>
<Button small title={'Log Out'} onPress={this.logout} />
</View>
</View>
:
<View style={[AppStyles.paddingHorizontal, AppStyles.paddingVerticalSml]}>
<Button small title={'Log In'} onPress={() => this.onPress(Actions.login)} />
</View>
}
</View>
</View>
</View>
)
}
/* Export Component ==================================================================== */
export default Menu;
|
src/client/components/todos/todoitem.js | fabriciocolombo/este | import PureComponent from '../../../lib/purecomponent';
import React from 'react';
import classnames from 'classnames';
import immutable from 'immutable';
import {deleteTodo} from '../../todos/actions';
export default class TodoItem extends PureComponent {
render() {
const todo = this.props.todo;
return (
<li className={classnames({editing: false})}>
<label>{todo.get('title')}</label>
<button onClick={() => deleteTodo(todo)}>x</button>
</li>
);
}
}
TodoItem.propTypes = {
todo: React.PropTypes.instanceOf(immutable.Map)
};
|
app/javascript/mastodon/components/column_header.js | glitch-soc/mastodon | import React from 'react';
import PropTypes from 'prop-types';
import { createPortal } from 'react-dom';
import classNames from 'classnames';
import { FormattedMessage, injectIntl, defineMessages } from 'react-intl';
import Icon from 'mastodon/components/icon';
const messages = defineMessages({
show: { id: 'column_header.show_settings', defaultMessage: 'Show settings' },
hide: { id: 'column_header.hide_settings', defaultMessage: 'Hide settings' },
moveLeft: { id: 'column_header.moveLeft_settings', defaultMessage: 'Move column to the left' },
moveRight: { id: 'column_header.moveRight_settings', defaultMessage: 'Move column to the right' },
});
export default @injectIntl
class ColumnHeader extends React.PureComponent {
static contextTypes = {
router: PropTypes.object,
};
static propTypes = {
intl: PropTypes.object.isRequired,
title: PropTypes.node,
icon: PropTypes.string,
active: PropTypes.bool,
multiColumn: PropTypes.bool,
extraButton: PropTypes.node,
showBackButton: PropTypes.bool,
children: PropTypes.node,
pinned: PropTypes.bool,
placeholder: PropTypes.bool,
onPin: PropTypes.func,
onMove: PropTypes.func,
onClick: PropTypes.func,
appendContent: PropTypes.node,
collapseIssues: PropTypes.bool,
};
state = {
collapsed: true,
animating: false,
};
historyBack = () => {
if (window.history && window.history.length === 1) {
this.context.router.history.push('/');
} else {
this.context.router.history.goBack();
}
}
handleToggleClick = (e) => {
e.stopPropagation();
this.setState({ collapsed: !this.state.collapsed, animating: true });
}
handleTitleClick = () => {
this.props.onClick();
}
handleMoveLeft = () => {
this.props.onMove(-1);
}
handleMoveRight = () => {
this.props.onMove(1);
}
handleBackClick = () => {
this.historyBack();
}
handleTransitionEnd = () => {
this.setState({ animating: false });
}
handlePin = () => {
if (!this.props.pinned) {
this.context.router.history.replace('/');
}
this.props.onPin();
}
render () {
const { title, icon, active, children, pinned, multiColumn, extraButton, showBackButton, intl: { formatMessage }, placeholder, appendContent, collapseIssues } = this.props;
const { collapsed, animating } = this.state;
const wrapperClassName = classNames('column-header__wrapper', {
'active': active,
});
const buttonClassName = classNames('column-header', {
'active': active,
});
const collapsibleClassName = classNames('column-header__collapsible', {
'collapsed': collapsed,
'animating': animating,
});
const collapsibleButtonClassName = classNames('column-header__button', {
'active': !collapsed,
});
let extraContent, pinButton, moveButtons, backButton, collapseButton;
if (children) {
extraContent = (
<div key='extra-content' className='column-header__collapsible__extra'>
{children}
</div>
);
}
if (multiColumn && pinned) {
pinButton = <button key='pin-button' className='text-btn column-header__setting-btn' onClick={this.handlePin}><Icon id='times' /> <FormattedMessage id='column_header.unpin' defaultMessage='Unpin' /></button>;
moveButtons = (
<div key='move-buttons' className='column-header__setting-arrows'>
<button title={formatMessage(messages.moveLeft)} aria-label={formatMessage(messages.moveLeft)} className='icon-button column-header__setting-btn' onClick={this.handleMoveLeft}><Icon id='chevron-left' /></button>
<button title={formatMessage(messages.moveRight)} aria-label={formatMessage(messages.moveRight)} className='icon-button column-header__setting-btn' onClick={this.handleMoveRight}><Icon id='chevron-right' /></button>
</div>
);
} else if (multiColumn && this.props.onPin) {
pinButton = <button key='pin-button' className='text-btn column-header__setting-btn' onClick={this.handlePin}><Icon id='plus' /> <FormattedMessage id='column_header.pin' defaultMessage='Pin' /></button>;
}
if (!pinned && (multiColumn || showBackButton)) {
backButton = (
<button onClick={this.handleBackClick} className='column-header__back-button'>
<Icon id='chevron-left' className='column-back-button__icon' fixedWidth />
<FormattedMessage id='column_back_button.label' defaultMessage='Back' />
</button>
);
}
const collapsedContent = [
extraContent,
];
if (multiColumn) {
collapsedContent.push(pinButton);
collapsedContent.push(moveButtons);
}
if (children || (multiColumn && this.props.onPin)) {
collapseButton = (
<button
className={collapsibleButtonClassName}
title={formatMessage(collapsed ? messages.show : messages.hide)}
aria-label={formatMessage(collapsed ? messages.show : messages.hide)}
aria-pressed={collapsed ? 'false' : 'true'}
onClick={this.handleToggleClick}
>
<i className='icon-with-badge'>
<Icon id='sliders' />
{collapseIssues && <i className='icon-with-badge__issue-badge' />}
</i>
</button>
);
}
const hasTitle = icon && title;
const component = (
<div className={wrapperClassName}>
<h1 className={buttonClassName}>
{hasTitle && (
<button onClick={this.handleTitleClick}>
<Icon id={icon} fixedWidth className='column-header__icon' />
{title}
</button>
)}
{!hasTitle && backButton}
<div className='column-header__buttons'>
{hasTitle && backButton}
{extraButton}
{collapseButton}
</div>
</h1>
<div className={collapsibleClassName} tabIndex={collapsed ? -1 : null} onTransitionEnd={this.handleTransitionEnd}>
<div className='column-header__collapsible-inner'>
{(!collapsed || animating) && collapsedContent}
</div>
</div>
{appendContent}
</div>
);
if (multiColumn || placeholder) {
return component;
} else {
// The portal container and the component may be rendered to the DOM in
// the same React render pass, so the container might not be available at
// the time `render()` is called.
const container = document.getElementById('tabs-bar__portal');
if (container === null) {
// The container wasn't available, force a re-render so that the
// component can eventually be inserted in the container and not scroll
// with the rest of the area.
this.forceUpdate();
return component;
} else {
return createPortal(component, container);
}
}
}
}
|
react-native-kit/profile.js | fromatlantis/nojs | import React from 'react';
import {
View,
Text,
Image,
Navigator,
StyleSheet,
StatusBar,
TouchableOpacity,
Platform,
Dimensions
} from 'react-native';
import {
createNavigator,
createNavigationContainer,
TabRouter,
addNavigationHelpers,
StackNavigator
} from 'react-navigation';
import Mlist from './mlist';
class Profile extends React.Component {
static navigationOptions = {
title: '信贷员列表',
}
constructor(props) {
super(props);
this.state = {
currentTab: 0
};
}
render() {
const { navigate } = this.props.navigation;
return (
<View style={styles.content}>
<View style={styles.listContainer}>
<TouchableOpacity onPress={() => navigate('Mlist')}>
<View style={styles.listItem}>
<View style={styles.listIcon}>
<Image source={require('./img/icon.png')}
style={styles.image} resizeMode='contain'/>
</View>
<View style={styles.listDes}>
<Text style={styles.des}>信贷员A</Text>
<Text style={styles.des}>最新采集1</Text>
</View>
</View>
</TouchableOpacity>
<TouchableOpacity onPress={() => navigate('Mlist')}>
<View style={styles.listItem}>
<View style={styles.listIcon}>
<Image source={require('./img/icon.png')}
style={styles.image}/>
</View>
<View style={styles.listDes}>
<Text style={styles.des}>信贷员A</Text>
<Text style={styles.des}>最新采集1</Text>
</View>
</View>
</TouchableOpacity>
</View>
</View>
);
}
}
export default Profile;
const styles = StyleSheet.create({
container: {
flex: 1
},
content: {
flex: 1,
paddingTop: Platform.OS === 'android' ? 0 : 20, //或者配置android的translucent为true,从物理顶端开始显示
},
barContainer: {
// 容器需要添加direction才能变成让子元素flex
flexDirection: 'row',
paddingTop: Platform.OS === 'android' ? 0 : 20, //或者配置android的translucent为true,从物理顶端开始显示
backgroundColor: 'rgba(0,0,255,.1)',
zIndex: 1 //注意图片的resizeMode并不会改变原有的占位,导致覆盖掉barContainer,事件无法起作用。推荐图片指定宽高,则不用加zIndex属性
},
cell: {
flex: 1,
height: 40,
justifyContent: 'center'
},
barLeft: {
textAlign: 'left'
},
barTitle: {
textAlign: 'center'
},
barRight: {
textAlign: 'right'
},
cellfixed: {
height: 40,
width: 80,
justifyContent: 'center',
paddingLeft: 8,
paddingRight: 8
},
listContainer: {
paddingLeft: 15
},
listItem: {
flexDirection: 'row',
height: 80,
borderBottomWidth:1,
borderColor: '#ebebeb',
},
listIcon: {
justifyContent: 'center',
alignItems: 'center'
},
image: {
width: 60,
height: 60
},
listDes: {
flex: 1,
justifyContent: 'center'
},
des: {
marginLeft: 10,
marginBottom: 5
},
tabBar: {
flexDirection:'row',
borderTopWidth: 1,
borderColor: '#ccc'
},
tabBarItem: {
flex: 1,
height: 50,
alignItems: 'center',
justifyContent: 'center'
},
tabBarItemPlus: {
height: 50,
width: 50,
borderColor:'#ccc',
borderWidth: 1,
borderRadius: 40,
alignItems: 'center',
justifyContent: 'center',
marginTop: -25,
backgroundColor:'#fff'
},
tabBarItemPlusText: {
fontSize: 20
},
tabBarText: {
//color:'#555'
},
tabBarTextCurrent: {
color:'red'
}
});
|
src/static/containers/Home/index.js | Seedstars/django-react-redux-base | import React from 'react';
import { push } from 'react-router-redux';
import { connect } from 'react-redux';
import PropTypes from 'prop-types';
import './style.scss';
import reactLogo from './images/react-logo.png';
import reduxLogo from './images/redux-logo.png';
class HomeView extends React.Component {
static propTypes = {
statusText: PropTypes.string,
userName: PropTypes.string,
dispatch: PropTypes.func.isRequired
};
static defaultProps = {
statusText: '',
userName: ''
};
goToProtected = () => {
this.props.dispatch(push('/protected'));
};
render() {
return (
<div className="container">
<div className="margin-top-medium text-center">
<img className="page-logo margin-bottom-medium"
src={reactLogo}
alt="ReactJs"
/>
<img className="page-logo margin-bottom-medium"
src={reduxLogo}
alt="Redux"
/>
</div>
<div className="text-center">
<h1>Django React Redux Demo</h1>
<h4>Hello, {this.props.userName || 'guest'}.</h4>
</div>
<div className="margin-top-medium text-center">
<p>Attempt to access some <a onClick={this.goToProtected}><b>protected content</b></a>.</p>
</div>
<div className="margin-top-medium">
{this.props.statusText ?
<div className="alert alert-info">
{this.props.statusText}
</div>
:
null
}
</div>
</div>
);
}
}
const mapStateToProps = (state) => {
return {
userName: state.auth.userName,
statusText: state.auth.statusText
};
};
export default connect(mapStateToProps)(HomeView);
export { HomeView as HomeViewNotConnected };
|
templates/rubix/relay/relay-seed/src/common/sidebar.js | jeffthemaximum/jeffline | import React from 'react';
import {
Sidebar, SidebarNav, SidebarNavItem,
SidebarControls, SidebarControlBtn,
LoremIpsum, Grid, Row, Col, FormControl,
Label, Progress, Icon,
SidebarDivider
} from '@sketchpixy/rubix';
import { Link, withRouter } from 'react-router';
@withRouter
class ApplicationSidebar extends React.Component {
handleChange(e) {
this._nav.search(e.target.value);
}
render() {
return (
<div>
<Grid>
<Row>
<Col xs={12}>
<FormControl type='text' placeholder='Search...' onChange={::this.handleChange} className='sidebar-search' style={{border: 'none', background: 'none', margin: '10px 0 0 0', borderBottom: '1px solid #666', color: 'white'}} />
<div className='sidebar-nav-container'>
<SidebarNav style={{marginBottom: 0}} ref={(c) => this._nav = c}>
{ /** Pages Section */ }
<div className='sidebar-header'>PAGES</div>
<SidebarNavItem glyph='icon-fontello-gauge' name='Home' href='/' />
</SidebarNav>
</div>
</Col>
</Row>
</Grid>
</div>
);
}
}
class DummySidebar extends React.Component {
render() {
return (
<Grid>
<Row>
<Col xs={12}>
<div className='sidebar-header'>DUMMY SIDEBAR</div>
<LoremIpsum query='1p' />
</Col>
</Row>
</Grid>
);
}
}
@withRouter
export default class SidebarContainer extends React.Component {
render() {
return (
<div id='sidebar'>
<div id='avatar'>
<Grid>
<Row className='fg-white'>
<Col xs={4} collapseRight>
<img src='/imgs/app/avatars/avatar0.png' width='40' height='40' />
</Col>
<Col xs={8} collapseLeft id='avatar-col'>
<div style={{top: 23, fontSize: 16, lineHeight: 1, position: 'relative'}}>Anna Sanchez</div>
<div>
<Progress id='demo-progress' value={30} color='#ffffff'/>
<a href='#'>
<Icon id='demo-icon' bundle='fontello' glyph='lock-5' />
</a>
</div>
</Col>
</Row>
</Grid>
</div>
<SidebarControls>
<SidebarControlBtn bundle='fontello' glyph='docs' sidebar={0} />
<SidebarControlBtn bundle='fontello' glyph='chat-1' sidebar={1} />
<SidebarControlBtn bundle='fontello' glyph='chart-pie-2' sidebar={2} />
<SidebarControlBtn bundle='fontello' glyph='th-list-2' sidebar={3} />
<SidebarControlBtn bundle='fontello' glyph='bell-5' sidebar={4} />
</SidebarControls>
<div id='sidebar-container'>
<Sidebar sidebar={0}>
<ApplicationSidebar />
</Sidebar>
<Sidebar sidebar={1}>
<DummySidebar />
</Sidebar>
<Sidebar sidebar={2}>
<DummySidebar />
</Sidebar>
<Sidebar sidebar={3}>
<DummySidebar />
</Sidebar>
<Sidebar sidebar={4}>
<DummySidebar />
</Sidebar>
</div>
</div>
);
}
}
|
internals/templates/containers/App/index.js | j921216063/chenya | /**
*
* App.react.js
*
* This component is the skeleton around the actual pages, and should only
* contain code that should be seen on all pages. (e.g. navigation bar)
*
* NOTE: while this component should technically be a stateless functional
* component (SFC), hot reloading does not currently support SFCs. If hot
* reloading is not a necessity for you then you can refactor it and remove
* the linting exception.
*/
import React from 'react';
export default class App extends React.PureComponent { // eslint-disable-line react/prefer-stateless-function
static propTypes = {
children: React.PropTypes.node,
};
render() {
return (
<div>
{React.Children.toArray(this.props.children)}
</div>
);
}
}
|
frontend/react-stack/react/aluraflix/src/components/Carousel/index.js | wesleyegberto/courses-projects | import React from 'react';
import { VideoCardGroupContainer, Title, ExtraLink } from './styles';
import VideoCard from './components/VideoCard';
import Slider, { SliderItem } from './components/Slider';
function VideoCardGroup({ ignoreFirstVideo, category }) {
const categoryTitle = category.titulo;
const categoryColor = category.cor;
const categoryExtraLink = category.link_extra;
const videos = category.videos;
return (
<VideoCardGroupContainer>
{categoryTitle && (
<>
<Title style={{ backgroundColor: categoryColor || 'red' }}>
{categoryTitle}
</Title>
{categoryExtraLink &&
<ExtraLink href={categoryExtraLink.url} target="_blank">
{categoryExtraLink.text}
</ExtraLink>
}
</>
)}
<Slider>
{videos.map((video, index) => {
if (ignoreFirstVideo && index === 0) {
return null;
}
return (
<SliderItem key={video.titulo}>
<VideoCard
videoTitle={video.titulo}
videoURL={video.url}
categoryColor={categoryColor}
/>
</SliderItem>
);
})}
</Slider>
</VideoCardGroupContainer>
);
}
export default VideoCardGroup;
|
js/app.js | agevelt/ssb-explorer | "use strict";
import React from 'react';
import ReactDOM from 'react-dom';
import JSONstat from "jsonstat";
import SortTable from "./sortTable.js";
import getJSONStatFromUrl from "./remotedata.js";
import dataset1128 from "./staticdata.js"
const rootId = "dataTable";
function getParameterByName(name, url) {
if (!url) {
url = window.location.href;
}
name = name.replace(/[\[\]]/g, "\\$&");
var regex = new RegExp("[?&]" + name + "(=([^&#]*)|&|#|$)"),
results = regex.exec(url);
if (!results) return null;
if (!results[2]) return '';
return decodeURIComponent(results[2].replace(/\+/g, " "));
}
function createTable() {
// If 'source' query parameter is present, we try to retrieve and display the response in a table.
let sourceUrl = getParameterByName('source');
console.log(sourceUrl);
if(sourceUrl){
getJSONStatFromUrl(sourceUrl,
(failureString)=> {
createErrorMessage(failureString)
},
(remoteJsonStatData)=> {
ReactDOM.render(<SortTable rows={remoteJsonStatData.rows}
cols={remoteJsonStatData.cols} />,
document.getElementById(rootId))
});
}
// If no source is present, then we use a predefined static data source.
else {
let staticJsonStatData = JSONstat(dataset1128).Dataset(0).toTable({type: "object"});
ReactDOM.render(
<SortTable rows={staticJsonStatData.rows}
cols={staticJsonStatData.cols} />,
document.getElementById(rootId)
);
}
}
function createErrorMessage(errorString){
ReactDOM.render(
<div> {errorString} </div>,
document.getElementById(rootId)
);
}
createTable(); |
docs/src/examples/collections/Table/Variations/TableExamplePadded.js | Semantic-Org/Semantic-UI-React | import React from 'react'
import { Table } from 'semantic-ui-react'
const TableExamplePadded = () => (
<Table padded>
<Table.Header>
<Table.Row>
<Table.HeaderCell>Name</Table.HeaderCell>
<Table.HeaderCell>Status</Table.HeaderCell>
<Table.HeaderCell>Notes</Table.HeaderCell>
</Table.Row>
</Table.Header>
<Table.Body>
<Table.Row>
<Table.Cell>John</Table.Cell>
<Table.Cell>Approved</Table.Cell>
<Table.Cell>
He is a very nice guy and I enjoyed talking to him on the telephone. I
hope we get to talk again.
</Table.Cell>
</Table.Row>
<Table.Row>
<Table.Cell>Jamie</Table.Cell>
<Table.Cell>Approved</Table.Cell>
<Table.Cell>
Jamie was not interested in purchasing our product.
</Table.Cell>
</Table.Row>
</Table.Body>
</Table>
)
export default TableExamplePadded
|
example/src/screens/Types.js | MattDavies/react-native-navigation | import React from 'react';
import { StyleSheet, View, Text, ScrollView, TouchableHighlight } from 'react-native';
import Row from '../components/Row';
class Types extends React.Component {
toggleDrawer = () => {
this.props.navigator.toggleDrawer({
side: 'left',
animated: true
});
};
pushScreen = () => {
this.props.navigator.push({
screen: 'example.Types.Push',
title: 'New Screen',
});
};
pushTopTabsScreen = () => {
this.props.navigator.push({
screen: 'example.Types.TopTabs',
title: 'Top Tabs',
topTabs: [{
screenId: 'example.Types.TopTabs.TabOne',
title: 'Tab One',
}, {
screenId: 'example.Types.TopTabs.TabTwo',
title: 'Tab Two',
}],
});
};
showModal = () => {
this.props.navigator.showModal({
screen: 'example.Types.Modal',
title: 'Modal',
});
};
showLightBox = () => {
this.props.navigator.showLightBox({
screen: "example.Types.LightBox",
passProps: {
title: 'LightBox',
content: 'Hey there, I\'m a light box screen :D',
onClose: this.dismissLightBox,
},
style: {
backgroundBlur: 'dark',
backgroundColor: 'rgba(0, 0, 0, 0.7)',
}
});
};
dismissLightBox = () => {
this.props.navigator.dismissLightBox();
};
showInAppNotification = () => {
this.props.navigator.showInAppNotification({
screen: 'example.Types.Notification',
});
};
render() {
return (
<ScrollView style={styles.container}>
<Row title={'Toggle Drawer'} onPress={this.toggleDrawer} />
<Row title={'Push Screen'} testID={'pushScreen'} onPress={this.pushScreen} />
<Row title={'Top Tabs Screen'} onPress={this.pushTopTabsScreen} platform={'android'} />
<Row title={'Show Modal'} onPress={this.showModal} />
<Row title={'Show Lightbox'} onPress={this.showLightBox} />
<Row title={'Show In-App Notification'} onPress={this.showInAppNotification} />
</ScrollView>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
},
row: {
height: 48,
paddingHorizontal: 16,
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'center',
borderBottomWidth: 1,
borderBottomColor: 'rgba(0, 0, 0, 0.054)',
},
text: {
fontSize: 16,
},
});
export default Types;
|
src/components/Disclaimer.js | orbitdb/orbit-web | 'use strict'
import React from 'react'
import PropTypes from 'prop-types'
import '../styles/Disclaimer.scss'
function Disclaimer ({ text }) {
return (
<div className='Disclaimer'>
<div className='content'>{text}</div>
</div>
)
}
Disclaimer.propTypes = {
text: PropTypes.string.isRequired
}
export default Disclaimer
|
src/images/IconResume.js | benjaminmodayil/modayilme | import React from 'react';
function Resume() {
return (
<svg
xmlns="http://www.w3.org/2000/svg"
xmlnsXlink="http://www.w3.org/1999/xlink"
width="48"
height="48"
className="font-sans"
>
<defs>
<path id="a" d="M0 0H48V48H0z" opacity="1"></path>
<mask id="c" maskContentUnits="userSpaceOnUse" maskUnits="userSpaceOnUse">
<path d="M0 0H48V48H0z"></path>
<use fill="#fff" xlinkHref="#a"></use>
</mask>
<mask id="d" maskContentUnits="userSpaceOnUse" maskUnits="userSpaceOnUse">
<path d="M0 0H48V48H0z"></path>
<use fill="#fff" xlinkHref="#b"></use>
</mask>
</defs>
<g mask="url(#c)">
<path fill="#E06D70" fillRule="evenodd" d="M44 27H4l-3-4 4-4h38l4 4-3 4z"></path>
<path
fill="#E6E6E6"
fillRule="evenodd"
d="M41 47H7a2 2 0 01-2-2V3a2 2 0 012-2h24l12 12v32a2 2 0 01-2 2z"
></path>
<path fill="#B3B3B3" fillRule="evenodd" d="M31 1v10a2 2 0 002 2h10L31 1z"></path>
<path
fill="#E06D70"
fillRule="evenodd"
d="M45 41H3a2 2 0 01-2-2V23h46v16a2 2 0 01-2 2z"
></path>
</g>
<text fontSize="0" transform="translate(7 28)">
<tspan
x="0"
y="8"
fill="#FFF"
fontSize="8"
fontWeight="700"
style={{
whiteSpace: 'pre',
WebkitTextTransform: 'none',
textTransform: 'none'
}}
>
RESUME
</tspan>
</text>
<g mask="url(#d)">
<path
fill="#72C472"
fillRule="evenodd"
d="M28.125 12.625H24.75V4.75a.375.375 0 00-.375-.375h-.75a.375.375 0 00-.375.375v7.875h-3.375a.375.375 0 00-.295.607l4.125 5.25a.375.375 0 00.59 0l4.125-5.25a.375.375 0 00-.295-.607z"
></path>
</g>
</svg>
);
}
export default Resume;
|
examples/files/react/classComponent.js | dabbott/react-native-express | import React from 'react'
import { Button, View } from 'react-native'
export default class MyComponent extends React.Component {
render() {
return (
<View>
<Button title="Press me!" color="#1ACDA5" />
</View>
)
}
}
|
src/svg-icons/editor/short-text.js | hai-cea/material-ui | import React from 'react';
import pure from 'recompose/pure';
import SvgIcon from '../../SvgIcon';
let EditorShortText = (props) => (
<SvgIcon {...props}>
<path d="M4 9h16v2H4zm0 4h10v2H4z"/>
</SvgIcon>
);
EditorShortText = pure(EditorShortText);
EditorShortText.displayName = 'EditorShortText';
EditorShortText.muiName = 'SvgIcon';
export default EditorShortText;
|
src/components/Dropzone.js | dpastoor/assignment-manager | /**
* Created by devin on 1/4/16.
*/
import React, { Component } from 'react';
import ReactDOM from 'react-dom';
import _ from 'lodash';
import {Card, List, ListItem} from 'material-ui';
import {Assignment} from './Assignment';
import Dropzone from 'react-dropzone';
import axios from 'axios';
import FileCloudDone from 'react-material-icons/icons/file/cloud-done';
export default class DZ extends React.Component {
constructor(props) {
super(props);
this.state = {
files: []
}
}
onDrop(files) {
console.log(files);
this.sendFile(files[0]);
this.setState({
files: files
})
}
sendFile(file) {
let wl = window.location;
let options = {
headers: {
'Content-Type': file.type
}
};
axios.put('http://localhost:8080/api' + wl.pathname + wl.search + '&user=' + window.localStorage._amtoken, file, options)
.then(function (result) {
console.log(result.config.data.name + " uploaded");
})
.catch(function (err) {
console.log(err);
});
}
render() {
return (
<div style={{
flexGrow: 1,
minWidth: '10vw',
overflowY: 'auto',
marginLeft: '10px'
}}>
<Dropzone
ref="dropzone"
onDrop={this.onDrop.bind(this)}
style= {{
width: '45vw',
height: '80vh', // need to adaptively adjust size better
borderWidth: 2,
borderColor: '#666',
borderStyle: 'dashed',
borderRadius: 5
}}
>
<div>
<div style={{
textAlign: 'center'
}}>
<h3>
Upload Drop Area
</h3>
</div>
<div>
The following files should uploaded:
<List>
<ListItem
leftIcon={<FileCloudDone />}
primaryText="Phoenix Project"
secondaryText=".phx file"
/>
<ListItem
leftIcon={<FileCloudDone />}
primaryText="Project folder with all required Rmarkdown Files"
secondaryText=".zip file"
/>
<ListItem
leftIcon={<FileCloudDone />}
primaryText="Project Report"
secondaryText=".pdf file"
/>
</List>
</div>
</div>
</Dropzone>
</div>
);
}
}
|
app/javascript/mastodon/features/ui/components/media_modal.js | salvadorpla/mastodon | import React from 'react';
import ReactSwipeableViews from 'react-swipeable-views';
import ImmutablePropTypes from 'react-immutable-proptypes';
import PropTypes from 'prop-types';
import Video from 'mastodon/features/video';
import ExtendedVideoPlayer from 'mastodon/components/extended_video_player';
import classNames from 'classnames';
import { defineMessages, injectIntl, FormattedMessage } from 'react-intl';
import IconButton from 'mastodon/components/icon_button';
import ImmutablePureComponent from 'react-immutable-pure-component';
import ImageLoader from './image_loader';
import Icon from 'mastodon/components/icon';
const messages = defineMessages({
close: { id: 'lightbox.close', defaultMessage: 'Close' },
previous: { id: 'lightbox.previous', defaultMessage: 'Previous' },
next: { id: 'lightbox.next', defaultMessage: 'Next' },
});
export const previewState = 'previewMediaModal';
export default @injectIntl
class MediaModal extends ImmutablePureComponent {
static propTypes = {
media: ImmutablePropTypes.list.isRequired,
status: ImmutablePropTypes.map,
index: PropTypes.number.isRequired,
onClose: PropTypes.func.isRequired,
intl: PropTypes.object.isRequired,
};
static contextTypes = {
router: PropTypes.object,
};
state = {
index: null,
navigationHidden: false,
};
handleSwipe = (index) => {
this.setState({ index: index % this.props.media.size });
}
handleNextClick = () => {
this.setState({ index: (this.getIndex() + 1) % this.props.media.size });
}
handlePrevClick = () => {
this.setState({ index: (this.props.media.size + this.getIndex() - 1) % this.props.media.size });
}
handleChangeIndex = (e) => {
const index = Number(e.currentTarget.getAttribute('data-index'));
this.setState({ index: index % this.props.media.size });
}
handleKeyDown = (e) => {
switch(e.key) {
case 'ArrowLeft':
this.handlePrevClick();
e.preventDefault();
e.stopPropagation();
break;
case 'ArrowRight':
this.handleNextClick();
e.preventDefault();
e.stopPropagation();
break;
}
}
componentDidMount () {
window.addEventListener('keydown', this.handleKeyDown, false);
if (this.context.router) {
const history = this.context.router.history;
history.push(history.location.pathname, previewState);
this.unlistenHistory = history.listen(() => {
this.props.onClose();
});
}
}
componentWillUnmount () {
window.removeEventListener('keydown', this.handleKeyDown);
if (this.context.router) {
this.unlistenHistory();
if (this.context.router.history.location.state === previewState) {
this.context.router.history.goBack();
}
}
}
getIndex () {
return this.state.index !== null ? this.state.index : this.props.index;
}
toggleNavigation = () => {
this.setState(prevState => ({
navigationHidden: !prevState.navigationHidden,
}));
};
handleStatusClick = e => {
if (e.button === 0 && !(e.ctrlKey || e.metaKey)) {
e.preventDefault();
this.context.router.history.push(`/statuses/${this.props.status.get('id')}`);
}
}
render () {
const { media, status, intl, onClose } = this.props;
const { navigationHidden } = this.state;
const index = this.getIndex();
let pagination = [];
const leftNav = media.size > 1 && <button tabIndex='0' className='media-modal__nav media-modal__nav--left' onClick={this.handlePrevClick} aria-label={intl.formatMessage(messages.previous)}><Icon id='chevron-left' fixedWidth /></button>;
const rightNav = media.size > 1 && <button tabIndex='0' className='media-modal__nav media-modal__nav--right' onClick={this.handleNextClick} aria-label={intl.formatMessage(messages.next)}><Icon id='chevron-right' fixedWidth /></button>;
if (media.size > 1) {
pagination = media.map((item, i) => {
const classes = ['media-modal__button'];
if (i === index) {
classes.push('media-modal__button--active');
}
return (<li className='media-modal__page-dot' key={i}><button tabIndex='0' className={classes.join(' ')} onClick={this.handleChangeIndex} data-index={i}>{i + 1}</button></li>);
});
}
const content = media.map((image) => {
const width = image.getIn(['meta', 'original', 'width']) || null;
const height = image.getIn(['meta', 'original', 'height']) || null;
if (image.get('type') === 'image') {
return (
<ImageLoader
previewSrc={image.get('preview_url')}
src={image.get('url')}
width={width}
height={height}
alt={image.get('description')}
key={image.get('url')}
onClick={this.toggleNavigation}
/>
);
} else if (image.get('type') === 'video') {
const { time } = this.props;
return (
<Video
preview={image.get('preview_url')}
blurhash={image.get('blurhash')}
src={image.get('url')}
width={image.get('width')}
height={image.get('height')}
startTime={time || 0}
onCloseVideo={onClose}
detailed
alt={image.get('description')}
key={image.get('url')}
/>
);
} else if (image.get('type') === 'gifv') {
return (
<ExtendedVideoPlayer
src={image.get('url')}
muted
controls={false}
width={width}
height={height}
key={image.get('preview_url')}
alt={image.get('description')}
onClick={this.toggleNavigation}
/>
);
}
return null;
}).toArray();
// you can't use 100vh, because the viewport height is taller
// than the visible part of the document in some mobile
// browsers when it's address bar is visible.
// https://developers.google.com/web/updates/2016/12/url-bar-resizing
const swipeableViewsStyle = {
width: '100%',
height: '100%',
};
const containerStyle = {
alignItems: 'center', // center vertically
};
const navigationClassName = classNames('media-modal__navigation', {
'media-modal__navigation--hidden': navigationHidden,
});
return (
<div className='modal-root__modal media-modal'>
<div
className='media-modal__closer'
role='presentation'
onClick={onClose}
>
<ReactSwipeableViews
style={swipeableViewsStyle}
containerStyle={containerStyle}
onChangeIndex={this.handleSwipe}
onSwitching={this.handleSwitching}
index={index}
>
{content}
</ReactSwipeableViews>
</div>
<div className={navigationClassName}>
<IconButton className='media-modal__close' title={intl.formatMessage(messages.close)} icon='times' onClick={onClose} size={40} />
{leftNav}
{rightNav}
{status && (
<div className={classNames('media-modal__meta', { 'media-modal__meta--shifted': media.size > 1 })}>
<a href={status.get('url')} onClick={this.handleStatusClick}><FormattedMessage id='lightbox.view_context' defaultMessage='View context' /></a>
</div>
)}
<ul className='media-modal__pagination'>
{pagination}
</ul>
</div>
</div>
);
}
}
|
server/priv/js/components/TimelineFilter.react.js | bks7/mzbench | import React from 'react';
import MZBenchRouter from '../utils/MZBenchRouter';
import MZBenchActions from '../actions/MZBenchActions';
import BenchStore from '../stores/BenchStore';
class TimelineFilter extends React.Component {
constructor(props) {
super(props);
this.autoSearchHandler = null;
this.state = {filter: this.props.filter};
}
componentWillReceiveProps(nextProps) {
if (this.props.filter != nextProps.filter) {
this.setState({filter: nextProps.filter});
}
}
render() {
return (
<form>
<div className="form-group">
<div className="input-group">
<div className="input-group-addon">Filter</div>
<input type="text" ref="filterInput" className="form-control" placeholder="Search Benchmarks" onKeyDown={this._onKeyDown.bind(this)} value={this.state.filter} onChange={this._onChange.bind(this)} />
</div>
</div>
</form>
);
}
_runSearch() {
MZBenchRouter.navigate("/timeline", {q: this.state.filter});
}
_onKeyDown(event) {
if (event.key === 'Enter') {
event.preventDefault();
this._runSearch();
}
}
_onChange(event) {
this.setState({filter: event.target.value});
if (this.autoSearchHandler) {
clearTimeout(this.autoSearchHandler);
}
this.autoSearchHandler = setTimeout(() => this._runSearch(), this.props.autoSearchInterval);
}
};
TimelineFilter.propTypes = {
filter: React.PropTypes.string,
autoSearchInterval: React.PropTypes.number
};
TimelineFilter.defaultProps = {
autoSearchInterval: 500
};
export default TimelineFilter;
|
docs/app/Examples/views/Statistic/Variations/Horizontal.js | ben174/Semantic-UI-React | import React from 'react'
import { Statistic } from 'semantic-ui-react'
const Horizontal = () => <Statistic horizontal value='2,204' label='Views' />
export default Horizontal
|
src/components/ShareModal/index.js | iris-dni/iris-frontend | import React from 'react';
import settings from 'settings';
import ModalIntro from 'components/ModalIntro';
import openShareWindow from 'helpers/sharing/openShareWindow';
import ShareModalButtons from 'components/ShareModalButtons';
const ShareModal = ({ title, intro, buttons }) => (
<div>
<ModalIntro
title={title}
intro={intro}
/>
<ShareModalButtons
openPopup={(url, event) => openShareWindow(url, event, settings.shareButtons.popupTitle)}
buttons={buttons}
/>
</div>
);
export default ShareModal;
|
front_end/front_end_app/src/client/auth/login.react.js | carlodicelico/horizon | import './login.styl';
import Component from '../components/component.react';
import React from 'react';
import exposeRouter from '../components/exposerouter.react';
import {focusInvalidField} from '../lib/validation';
@exposeRouter
export default class Login extends Component {
static propTypes = {
actions: React.PropTypes.object.isRequired,
auth: React.PropTypes.object.isRequired,
msg: React.PropTypes.object.isRequired,
router: React.PropTypes.func
}
onFormSubmit(e) {
e.preventDefault();
const {actions: {auth}, auth: {form}} = this.props;
auth.login(form.fields)
.then(() => this.redirectAfterLogin())
.catch(focusInvalidField(this));
}
redirectAfterLogin() {
const {router} = this.props;
const nextPath = router.getCurrentQuery().nextPath;
router.replaceWith(nextPath || 'home');
}
render() {
const {
actions: {auth: actions},
auth: {form},
msg: {auth: {form: msg}}
} = this.props;
return (
<div className="login">
<form onSubmit={::this.onFormSubmit}>
<fieldset disabled={form.disabled}>
<legend>{msg.legend}</legend>
<input
autoFocus
name="email"
onChange={actions.setFormField}
placeholder={msg.placeholder.email}
value={form.fields.email}
/>
<br />
<input
name="password"
onChange={actions.setFormField}
placeholder={msg.placeholder.password}
type="password"
value={form.fields.password}
/>
<br />
<button
children={msg.button.login}
type="submit"
/>
{form.error &&
<span className="error-message">{form.error.message}</span>
}
<div>{msg.hint}</div>
</fieldset>
</form>
</div>
);
}
}
|
src/mobile/components/MainView/index.js | u-wave/web | import React from 'react';
import PropTypes from 'prop-types';
import { useTranslator } from '@u-wave/react-translate';
import AppBar from '@mui/material/AppBar';
import Toolbar from '@mui/material/Toolbar';
import Typography from '@mui/material/Typography';
import IconButton from '@mui/material/IconButton';
import HistoryIcon from '@mui/icons-material/History';
import MenuIcon from '@mui/icons-material/Menu';
import SongTitle from '../../../components/SongTitle';
import Video from '../../containers/Video';
import Chat from '../../containers/Chat';
import DrawerMenu from '../../containers/DrawerMenu';
import UsersDrawer from '../../containers/UsersDrawer';
import VideoDisabledMessage from './VideoDisabledMessage';
const waitlistIconStyle = {
fontSize: '125%',
textAlign: 'center',
};
const getWaitlistLabel = (size, position) => {
if (size > 0) {
const posText = position !== -1
? `${position + 1}/${size}`
: size;
return posText;
}
return '0';
};
function MainView({
media,
videoEnabled,
waitlistPosition,
waitlistSize,
onOpenRoomHistory,
onOpenDrawer,
onOpenWaitlist,
onEnableVideo,
}) {
const { t } = useTranslator();
let title = t('booth.empty');
if (media) {
title = <SongTitle artist={media.artist} title={media.title} />;
}
return (
<div className="MainView">
<AppBar position="static" className="MainView-appBar">
<Toolbar>
<IconButton aria-label="Menu" onClick={onOpenDrawer}>
<MenuIcon />
</IconButton>
<Typography variant="h6" className="MainView-title">
{title}
</Typography>
<IconButton onClick={onOpenRoomHistory}>
<HistoryIcon />
</IconButton>
<IconButton style={waitlistIconStyle} onClick={onOpenWaitlist}>
{getWaitlistLabel(waitlistSize, waitlistPosition)}
</IconButton>
</Toolbar>
</AppBar>
<div className="MainView-content">
<div className="MobileApp-video">
<Video
enabled={videoEnabled}
size="large"
/>
{!videoEnabled && (
<VideoDisabledMessage onEnableVideo={onEnableVideo} />
)}
</div>
<div className="MobileApp-chat">
<Chat />
</div>
</div>
<DrawerMenu />
<UsersDrawer />
</div>
);
}
MainView.propTypes = {
media: PropTypes.object,
videoEnabled: PropTypes.bool.isRequired,
waitlistPosition: PropTypes.number.isRequired,
waitlistSize: PropTypes.number.isRequired,
onOpenRoomHistory: PropTypes.func.isRequired,
onOpenWaitlist: PropTypes.func.isRequired,
onOpenDrawer: PropTypes.func.isRequired,
onEnableVideo: PropTypes.func.isRequired,
};
export default MainView;
|
src/Illuminate/Foundation/Console/Presets/react-stubs/Example.js | reinink/framework | import React, { Component } from 'react';
import ReactDOM from 'react-dom';
export default class Example extends Component {
render() {
return (
<div className="container">
<div className="row">
<div className="col-md-8 col-md-offset-2">
<div className="panel panel-default">
<div className="panel-heading">Example Component</div>
<div className="panel-body">
I'm an example component!
</div>
</div>
</div>
</div>
</div>
);
}
}
if (document.getElementById('example')) {
ReactDOM.render(<Example />, document.getElementById('example'));
}
|
fields/types/url/UrlColumn.js | brianjd/keystone | import React from 'react';
import ItemsTableCell from '../../components/ItemsTableCell';
import ItemsTableValue from '../../components/ItemsTableValue';
var UrlColumn = React.createClass({
displayName: 'UrlColumn',
propTypes: {
col: React.PropTypes.object,
data: React.PropTypes.object,
},
renderValue () {
var value = this.props.data.fields[this.props.col.path];
if (!value) return;
// if the value doesn't start with a prototcol, assume http for the href
var href = value;
if (href && !/^(mailto\:)|(\w+\:\/\/)/.test(href)) {
href = 'http://' + value;
}
// strip the protocol from the link if it's http(s)
var label = value.replace(/^https?\:\/\//i, '');
return (
<ItemsTableValue to={href} padded exterior field={this.props.col.type}>
{label}
</ItemsTableValue>
);
},
render () {
return (
<ItemsTableCell>
{this.renderValue()}
</ItemsTableCell>
);
},
});
module.exports = UrlColumn;
|
ReactApp/src/Cards/Tickets/OnSiteCard.js | hoh/FolkMarsinne | 'use strict';
import React from 'react';
import {Card, CardActions, CardHeader, CardMedia, CardTitle, CardText} from 'material-ui/Card';
import {Table, TableBody, TableHeader, TableHeaderColumn, TableRow, TableRowColumn} from 'material-ui/Table';
import Paper from 'material-ui/Paper';
const i18n_strings = {
fr: {
title: 'Sur Place',
intro: (<div>
<p>
Les tickets pour <b>une journée</b> sont à acheter sur place
à l'entrée du festival. Les <b>Pass 3 jours</b> y seront également
disponibles, mais à un tarif plus élevé qu'en prévente.
</p>
<p>
Seuls les paiements en <b>espèces</b> seront acceptés pour le
paiement sur place, <b>PAS de paiement par carte bancaire ou
par chèque</b>. Si vous pouvez prévoir la monnaie exacte, c'est
encore mieux.
</p>
</div>),
table: {
friday: 'Vendredi',
saturday: 'Samedi',
sunday: 'Dimanche',
normal_fare: 'Tarif Normal',
fare_12_25: 'Tarif 12-25 ans',
pass_3_days: 'Pass 3 jours',
evening: 'Soirée',
},
free_for_kids: 'Entrée gratuite pour les moins de 12 ans accompagnés d\'un adulte responsable.',
notes_title: 'Remarques',
notes: (
<ul>
<li>
Vous pourrez toujours acheter vos tickets sur place aux tarifs
indiqués ci-dessus — il y en aura pour tout le monde.
</li>
<li>
Le distributeur de billets de banque le plus proche se trouve à 1.7 km,
au <a href="http://www.bpost2.be/locations/zip_res/fr/map_zone_ins.php?ins=61028&word=couthuin">bureau de poste de Couthuin</a>:
<p>
Rue Sur Les Trixhes 3, 4216 Couthuin
<a href="https://www.google.be/maps/place/bpost+SA/@50.5285247,5.1353813,17z/data=!4m5!3m4!1s0x0000000000000000:0xc19cd0daccb7ea38!8m2!3d50.5285473!4d5.1383507">
(Google Maps)
</a>
</p>
</li>
</ul>
),
},
en: {
title: 'On Site',
intro: (<div>
<p>
<b>One day</b> tickets will be available at the entry of the festival
only. <b>3 days Pass</b> will also be available on site, but at a higher
price than pre-sales.
</p>
<p>
Only <b>cash</b> payments will be available on site, <b>NOR cards
or bank cheque</b>. If you have the exact amount, that’s even better !
</p>
</div>),
table: {
friday: 'Friday',
saturday: 'Saturday',
sunday: 'Sunday',
normal_fare: 'Normal fare',
fare_12_25: '12-25 yo',
pass_3_days: 'Pass 3 days',
evening: 'Evening',
},
free_for_kids: 'Free entrance for children until 12 year, with a responsible adult.',
notes_title: 'Notes',
notes: (
<ul>
<li>
Tickets will always be available on site — there will be enough for
everyone.
</li>
<li>
The closest ATM is 1.7 km away,
at <a href="http://www.bpost2.be/locations/zip_res/fr/map_zone_ins.php?ins=61028&word=couthuin">bureau de poste de Couthuin</a>:
<p>
Rue Sur Les Trixhes 3, 4216 Couthuin
<a href="https://www.google.be/maps/place/bpost+SA/@50.5285247,5.1353813,17z/data=!4m5!3m4!1s0x0000000000000000:0xc19cd0daccb7ea38!8m2!3d50.5285473!4d5.1383507">
(Google Maps)
</a>
</p>
</li>
</ul>
),
},
nl: {
title: 'Ter plaatse',
intro: (<div>
<p>
<b>Een dag</b> tickets zijn rechtstreeks op de site te kopen.
De <b>3 dagen Pass</b> zullen daar ook beschikbaar zijn maar op hooger prijs dan in voorverkoop.
</p>
<p>
Aandacht! Ter plaatse kan <b>NIET met bankkaart of met bankcheques</b> betaald worden. Probeer ook met pasmunt te betalen.
</p>
</div>),
table: {
friday: 'Vrijdag',
saturday: 'Zaterdag',
sunday: 'Zondag',
normal_fare: 'Gewoon',
fare_12_25: '12-25 jaar',
pass_3_days: '3 dagen',
evening: 'Avondtarief',
},
free_for_kids: 'Gratis voor kinderen jonger dan 12 jaar, die vergezeld worden door een verantwoordelijke volwassene.',
notes_title: 'Opmerkingen',
notes: (
<ul>
<li>
Heb je geen ticket in voorverkoop kunnen nemen, dan kan je tickets ter
plaatse kopen op tarief hierboven vermeld - er zullen er voor iedereen beschikbaar zijn!
</li>
<li>
De dichtstbijzijnde geldautomaat is 1,7 km:
<a href="http://www.bpost2.be/locations/zip_res/fr/map_zone_ins.php?ins=61028&word=couthuin">bureau de poste de Couthuin</a>:
<p>
Rue Sur Les Trixhes 3, 4216 Couthuin
<a href="https://www.google.be/maps/place/bpost+SA/@50.5285247,5.1353813,17z/data=!4m5!3m4!1s0x0000000000000000:0xc19cd0daccb7ea38!8m2!3d50.5285473!4d5.1383507">
(Google Maps)
</a>
</p>
</li>
</ul>
),
},
}
export default class EventsCard extends React.Component {
render() {
var strings = i18n_strings[this.props.lang] || i18n_strings['fr'];
return (
<Card>
<CardTitle title={strings.title} id="onsite" />
<CardText>
{strings.intro}
<Paper zDepth={2}>
<Table >
<TableHeader displaySelectAll={false} adjustForCheckbox={false}>
<TableRow>
<TableHeaderColumn></TableHeaderColumn>
<TableHeaderColumn>{strings.table.normal_fare}</TableHeaderColumn>
<TableHeaderColumn>{strings.table.fare_12_25}</TableHeaderColumn>
<TableHeaderColumn>{strings.table.evening}</TableHeaderColumn>
</TableRow>
</TableHeader>
<TableBody displayRowCheckbox={false}>
<TableRow>
<TableRowColumn>{strings.table.pass_3_days}</TableRowColumn>
<TableRowColumn>57 €</TableRowColumn>
<TableRowColumn>47 €</TableRowColumn>
<TableRowColumn>/</TableRowColumn>
</TableRow>
<TableRow>
<TableRowColumn>{strings.table.friday}</TableRowColumn>
<TableRowColumn>17 €</TableRowColumn>
<TableRowColumn>14 €</TableRowColumn>
<TableRowColumn>/</TableRowColumn>
</TableRow>
<TableRow>
<TableRowColumn>{strings.table.saturday}</TableRowColumn>
<TableRowColumn>33 €</TableRowColumn>
<TableRowColumn>27 €</TableRowColumn>
<TableRowColumn>15 € (20h30)</TableRowColumn>
</TableRow>
<TableRow>
<TableRowColumn>{strings.table.sunday}</TableRowColumn>
<TableRowColumn>22 €</TableRowColumn>
<TableRowColumn>18 €</TableRowColumn>
<TableRowColumn>10 € (19h00)</TableRowColumn>
</TableRow>
</TableBody>
</Table>
</Paper>
<p><i>
{strings.free_for_kids}
</i></p>
<h3>{strings.notes_title}</h3>
{strings.notes}
</CardText>
</Card>
);
}
}
|
src/parser/druid/restoration/modules/talents/Flourish.js | FaideWW/WoWAnalyzer | import React from 'react';
import { STATISTIC_ORDER } from 'interface/others/StatisticBox';
import StatisticBox from 'interface/others/StatisticBox';
import { formatPercentage, formatNumber } from 'common/format';
import calculateEffectiveHealing from 'parser/core/calculateEffectiveHealing';
import SpellIcon from 'common/SpellIcon';
import SpellLink from 'common/SpellLink';
import SPELLS from 'common/SPELLS';
import Analyzer from 'parser/core/Analyzer';
import HotTracker from '../core/hottracking/HotTracker';
import { HOTS_AFFECTED_BY_ESSENCE_OF_GHANIR } from '../../constants';
const debug = false;
const FLOURISH_EXTENSION = 8000;
const FLOURISH_HEALING_INCREASE = 1;
/*
Extends the duration of all of your heal over time effects on friendly targets within 60 yards by 8 sec,
and increases the rate of your heal over time effects by 100% for 8 sec.
*/
// TODO: Idea - Give suggestions on low amount/duration extended with flourish on other HoTs
class Flourish extends Analyzer {
static dependencies = {
hotTracker: HotTracker,
};
// Counters for hot extension
flourishCount = 0;
flourishes = [];
wgsExtended = 0; // tracks how many flourishes extended Wild Growth
cwsExtended = 0; // tracks how many flourishes extended Cenarion Ward
tranqsExtended = 0;
hasCenarionWard = false;
rejuvCount = 0;
wgCount = 0;
lbCount = 0;
regrowthCount = 0;
sbCount = 0;
cultCount = 0;
tranqCount = 0;
groveTendingCount = 0;
// Counters for increased ticking rate of hots
increasedRateTotalHealing = 0;
increasedRateRejuvenationHealing = 0;
increasedRateWildGrowthHealing = 0;
increasedRateCenarionWardHealing = 0;
increasedRateCultivationHealing = 0;
increasedRateLifebloomHealing = 0;
increasedRateRegrowthHealing = 0;
increasedRateTranqHealing = 0;
increasedRateGroveTendingHealing = 0;
constructor(...args) {
super(...args);
this.active = this.selectedCombatant.hasTalent(SPELLS.FLOURISH_TALENT.id);
this.hasCenarionWard = this.selectedCombatant.hasTalent(SPELLS.CENARION_WARD_TALENT.id);
}
on_byPlayer_heal(event) {
const spellId = event.ability.guid;
if (this.selectedCombatant.hasBuff(SPELLS.FLOURISH_TALENT.id) && HOTS_AFFECTED_BY_ESSENCE_OF_GHANIR.includes(spellId)) {
switch (spellId) {
case SPELLS.REJUVENATION.id:
this.increasedRateRejuvenationHealing += calculateEffectiveHealing(event, FLOURISH_HEALING_INCREASE);
break;
case SPELLS.REJUVENATION_GERMINATION.id:
this.increasedRateRejuvenationHealing += calculateEffectiveHealing(event, FLOURISH_HEALING_INCREASE);
break;
case SPELLS.WILD_GROWTH.id:
this.increasedRateWildGrowthHealing += calculateEffectiveHealing(event, FLOURISH_HEALING_INCREASE);
break;
case SPELLS.CENARION_WARD_HEAL.id:
this.increasedRateCenarionWardHealing += calculateEffectiveHealing(event, FLOURISH_HEALING_INCREASE);
break;
case SPELLS.CULTIVATION.id:
this.increasedRateCultivationHealing += calculateEffectiveHealing(event, FLOURISH_HEALING_INCREASE);
break;
case SPELLS.LIFEBLOOM_HOT_HEAL.id:
this.increasedRateLifebloomHealing += calculateEffectiveHealing(event, FLOURISH_HEALING_INCREASE);
break;
case SPELLS.GROVE_TENDING.id:
this.increasedRateGroveTendingHealing += calculateEffectiveHealing(event, FLOURISH_HEALING_INCREASE);
break;
case SPELLS.REGROWTH.id:
if (event.tick === true) {
this.increasedRateRegrowthHealing += calculateEffectiveHealing(event, FLOURISH_HEALING_INCREASE);
}
break;
case SPELLS.TRANQUILITY_HEAL.id:
if (event.tick === true) {
this.increasedRateTranqHealing += calculateEffectiveHealing(event, FLOURISH_HEALING_INCREASE);
}
break;
default:
console.error('EssenceOfGhanir: Error, could not identify this object as a HoT: %o', event);
}
if ((SPELLS.REGROWTH.id === spellId || SPELLS.TRANQUILITY_HEAL.id) && event.tick !== true) {
return;
}
this.increasedRateTotalHealing += calculateEffectiveHealing(event, FLOURISH_HEALING_INCREASE);
}
}
on_byPlayer_cast(event) {
const spellId = event.ability.guid;
if (SPELLS.FLOURISH_TALENT.id !== spellId) {
return;
}
this.flourishCount += 1;
debug && console.log(`Flourish cast #: ${this.flourishCount}`);
const newFlourish = {
name: `Flourish #${this.flourishCount}`,
healing: 0,
masteryHealing: 0,
procs: 0,
duration: 0,
};
this.flourishes.push(newFlourish);
let foundWg = false;
let foundCw = false;
let foundTranq = false;
Object.keys(this.hotTracker.hots).forEach(playerId => {
Object.keys(this.hotTracker.hots[playerId]).forEach(spellIdString => {
const spellId = Number(spellIdString);
// due to flourish's refresh mechanc, we don't include it in Flourish numbers
const attribution = spellId === SPELLS.CULTIVATION.id ? null : newFlourish;
this.hotTracker.addExtension(attribution, FLOURISH_EXTENSION, playerId, spellId);
if (spellId === SPELLS.WILD_GROWTH.id) {
foundWg = true;
this.wgCount += 1;
} else if (spellId === SPELLS.CENARION_WARD_HEAL.id) {
foundCw = true;
} else if (spellId === SPELLS.REJUVENATION.id || spellId === SPELLS.REJUVENATION_GERMINATION.id) {
this.rejuvCount += 1;
} else if (spellId === SPELLS.REGROWTH.id) {
this.regrowthCount += 1;
} else if (spellId === SPELLS.LIFEBLOOM_HOT_HEAL.id) {
this.lbCount += 1;
} else if (spellId === SPELLS.SPRING_BLOSSOMS.id) {
this.sbCount += 1;
} else if (spellId === SPELLS.CULTIVATION.id) {
this.cultCount += 1;
} else if (spellId === SPELLS.GROVE_TENDING.id) {
this.groveTendingCount += 1;
} else if (spellId === SPELLS.TRANQUILITY_HEAL.id) {
foundTranq = true;
this.tranqCount += 1;
}
});
});
if (foundWg) {
this.wgsExtended += 1;
}
if (foundCw) {
this.cwsExtended += 1;
}
if (foundTranq) {
this.tranqsExtended += 1;
}
}
get totalExtensionHealing() {
return this.flourishes.reduce((acc, flourish) => acc + flourish.healing + flourish.masteryHealing, 0);
}
get averageHealing() {
return this.flourishCount === 0 ? 0 : this.totalExtensionHealing / this.flourishCount;
}
get percentWgsExtended() {
return this.flourishCount === 0 ? 0 : this.wgsExtended / this.flourishCount;
}
get wildGrowthSuggestionThresholds() {
return {
actual: this.percentWgsExtended,
isLessThan: {
minor: 1.00,
average: 0.75,
major: 0.50,
},
style: 'percentage',
};
}
get percentCwsExtended() {
return (this.cwsExtended / this.flourishCount) || 0;
}
get cenarionWardSuggestionThresholds() {
return {
actual: this.percentCwsExtended,
isLessThan: {
minor: 1.00,
average: 0.00,
major: 0.00,
},
style: 'percentage',
};
}
suggestions(when) {
if(this.flourishCount === 0) {
return;
}
when(this.wildGrowthSuggestionThresholds)
.addSuggestion((suggest, actual, recommended) => {
return suggest(<>Your <SpellLink id={SPELLS.FLOURISH_TALENT.id} /> should always aim to extend a <SpellLink id={SPELLS.WILD_GROWTH.id} /></>)
.icon(SPELLS.FLOURISH_TALENT.icon)
.actual(`${formatPercentage(this.wgsExtended / this.flourishCount, 0)}% WGs extended.`)
.recommended(`${formatPercentage(recommended)}% is recommended`);
});
if(this.hasCenarionWard) {
when(this.cenarionWardSuggestionThresholds)
.addSuggestion((suggest, actual, recommended) => {
return suggest(<>Your <SpellLink id={SPELLS.FLOURISH_TALENT.id} /> should always aim to extend a <SpellLink id={SPELLS.CENARION_WARD_HEAL.id} /></>)
.icon(SPELLS.FLOURISH_TALENT.icon)
.actual(`${this.cwsExtended}/${this.flourishCount} CWs extended.`)
.recommended(`${formatPercentage(recommended)}% is recommended`);
});
}
}
statistic() {
const extendPercent = this.owner.getPercentageOfTotalHealingDone(this.totalExtensionHealing);
const increasedRatePercent = this.owner.getPercentageOfTotalHealingDone(this.increasedRateTotalHealing);
const totalPercent = this.owner.getPercentageOfTotalHealingDone(this.totalExtensionHealing + this.increasedRateTotalHealing);
return(
<StatisticBox
icon={<SpellIcon id={SPELLS.FLOURISH_TALENT.id} />}
value={`${formatPercentage(totalPercent)} %`}
label="Flourish Healing"
tooltip={`
The HoT extension contributed: <b>${formatPercentage(extendPercent)} %</b><br>
The HoT increased tick rate contributed: <b>${formatPercentage(increasedRatePercent)} %</b><br>
<ul>
${this.wildGrowth === 0 ? '' :
`<li>${formatPercentage(this.owner.getPercentageOfTotalHealingDone(this.increasedRateWildGrowthHealing))}% from Wild Growth</li>`}
${this.rejuvenation === 0 ? '' :
`<li>${formatPercentage(this.owner.getPercentageOfTotalHealingDone(this.increasedRateRejuvenationHealing))}% from Rejuvenation</li>`}
${this.cenarionWard === 0 ? '' :
`<li>${formatPercentage(this.owner.getPercentageOfTotalHealingDone(this.increasedRateCenarionWardHealing))}% from Cenarion Ward</li>`}
${this.lifebloom === 0 ? '' :
`<li>${formatPercentage(this.owner.getPercentageOfTotalHealingDone(this.increasedRateLifebloomHealing))}% from Lifebloom</li>`}
${this.regrowth === 0 ? '' :
`<li>${formatPercentage(this.owner.getPercentageOfTotalHealingDone(this.increasedRateRegrowthHealing))}% from Regrowth</li>`}
${this.cultivation === 0 ? '' :
`<li>${formatPercentage(this.owner.getPercentageOfTotalHealingDone(this.increasedRateCultivationHealing))}% from Cultivation</li>`}
${this.traquility === 0 ? '' :
`<li>${formatPercentage(this.owner.getPercentageOfTotalHealingDone(this.increasedRateTranqHealing))}% from Tranquillity</li>`}
${this.groveTending === 0 ? '' :
`<li>${formatPercentage(this.owner.getPercentageOfTotalHealingDone(this.increasedRateGroveTendingHealing))}% from Grove Tending</li>`}
</ul>
The per Flourish amounts do <i>not</i> include Cultivation due to its refresh mechanic.<br>
Your ${this.flourishCount} Flourish casts extended:
<ul>
<li>${this.wgsExtended}/${this.flourishCount} Wild Growth casts (${this.wgCount} HoTs)</li>
${this.hasCenarionWard
? `<li>${this.cwsExtended}/${this.flourishCount} Cenarion Wards</li>`
: ``
}
${this.rejuvCount > 0
? `<li>${this.rejuvCount} Rejuvenations</li>`
: ``
}
${this.regrowthCount > 0
? `<li>${this.regrowthCount} Regrowths</li>`
: ``
}
${this.lbCount > 0
? `<li>${this.lbCount} Lifeblooms</li>`
: ``
}
${this.sbCount > 0
? `<li>${this.sbCount} Spring Blossoms</li>`
: ``
}
${this.cultCount > 0
? `<li>${this.cultCount} Cultivations (not counted in HoT count and HoT healing totals)</li>`
: ``
}
${this.tranqCount > 0
? `<li>${this.tranqsExtended}/${this.flourishCount} Tranquillities casts (${this.tranqCount} HoTs)</li>`
: ``
}
${this.groveTendingCount > 0
? `<li>${this.groveTendingCount}/${this.flourishCount} Grove tendings</li>`
: ``
}
</ul>
<br>
The Healing column shows how much additional healing was done by the 8 extra seconds of HoT time. Note that if you Flourished near the end of a fight, numbers might be lower than you expect because extension healing isn't tallied until a HoT falls.`
}
>
<table className="table table-condensed">
<thead>
<tr>
<th>Cast</th>
<th># of HoTs</th>
<th>Healing</th>
</tr>
</thead>
<tbody>
{
this.flourishes.map((flourish, index) => (
<tr key={index}>
<th scope="row">{ index + 1 }</th>
<td>{ flourish.procs }</td>
<td>{ formatNumber(flourish.healing + flourish.masteryHealing) }</td>
</tr>
))
}
</tbody>
</table>
</StatisticBox>
);
}
statisticOrder = STATISTIC_ORDER.OPTIONAL();
}
export default Flourish;
|
src/modules/annotations/components/AnnotationsList/AnnotationsList.js | cltk/cltk_frontend | import React from 'react';
import PropTypes from 'prop-types';
import autoBind from 'react-autobind';
import RaisedButton from 'material-ui/RaisedButton';
import FlatButton from 'material-ui/FlatButton';
import './AnnotationsList.css';
class AnnotationsList extends React.Component {
constructor(props) {
super(props);
this.state = {
sortMethod: 'votes',
};
autoBind(this);
}
addDiscussionComment() {
$(this.newCommentForm).find('textarea').val('');
}
sortMethodSelect(value) {
this.setState({
sortMethod: value,
})
}
render() {
const { annotations } = this.props;
let discussionWrapClass = 'discussion-wrap';
if (this.state.discussionVisible) {
discussionWrapClass += ' discussion-visible';
}
let textareaPlaceholder = '';
if (currentUser) {
textareaPlaceholder = 'Enter your comment here . . .';
} else {
textareaPlaceholder = 'Please login to enter a comment.';
}
const sortSelectedLabelStyle = {
color: '#FFFFFF',
};
return (
<div className={discussionWrapClass}>
<div className="discussion-thread">
<div className="add-comment-wrap paper-shadow ">
<form
ref={(component) => { this.newCommentForm = component; }}
className="new-comment-form"
name="new-comment-form"
>
<div className="add-comment-row-1">
<div className="profile-picture paper-shadow">
<img
src={currentUser && currentUser.avatar ?
currentUser.avatar.url : '/images/default_user.jpg'}
alt="User"
/>
</div>
<textarea
className="new-comment-text"
name="newCommentText"
placeholder={textareaPlaceholder}
/>
</div>
<div className="add-comment-row-2 add-comment-row">
<div className="error-message">
<span className="error-message-text">Please enter your text to submit.</span>
</div>
{ currentUser ?
<RaisedButton
label="Submit"
className="submit-comment-button paper-shadow"
onClick={this.addDiscussionComment}
/>
:
<div
className="new-comment-login"
>
<FlatButton
label="Join"
className="join-link"
href="/sign-up"
/>
<FlatButton
label="Login"
className="login-link"
href="/sign-in"
/>
</div>
}
</div>
</form>
</div>
<div
className="sort-by-wrap"
>
{/*
<span className="sort-by-label">Sort by:</span>
<RaisedButton
label="Top"
className="sort-by-option selected-sort sort-by-top"
onClick={this.toggleSort}>
</RaisedButton>
<RaisedButton
label="Newest"
className="sort-by-option sort-by-new"
onClick={this.toggleSort}>
</RaisedButton>
*/}
</div>
{annotations && annotations.length === 0 ?
<div className="no-results-wrap">
<span className="no-results-text">No annotations.</span>
</div>
: ''
}
<div className="sort-method-select">
<FlatButton
label="Top votes"
labelStyle={this.state.sortMethod === 'votes' ? sortSelectedLabelStyle : {}}
backgroundColor={this.state.sortMethod === 'votes' ? '#795548' : ''}
onClick={this.sortMethodSelect.bind(null, 'votes')}
/>
<FlatButton
label="Recent"
labelStyle={this.state.sortMethod === 'recent' ? sortSelectedLabelStyle : {}}
backgroundColor={this.state.sortMethod === 'recent' ? '#795548' : ''}
onClick={this.sortMethodSelect.bind(null, 'recent')}
/>
</div>
{annotations && annotations.map((annotation, i) =>
<AnnotationItem
key={i}
className="discussion-comment paper-shadow"
annotation={annotation}
currentUser={currentUser}
/>
)}
</div>
</div>
);
}
}
AnnotationsList.propTypes = {
text: PropTypes.object.isRequired,
};
export default AnnotationsList;
|
src/checkAPI.js | HsuTing/cat-components | 'use strict';
import React from 'react';
import checkGlobal from 'utils/checkGlobal';
export default (
name,
func,
getData = callback => callback({}),
defaultData = {}
) => Component => {
const state = {};
state[`${name}CanUse`] = false;
return class CheckAPI extends React.Component {
constructor(props) {
super(props);
this.state = {
...state,
...defaultData
};
}
componentDidMount() {
checkGlobal.add(
name, func,
() => getData(
data => {
state[`${name}CanUse`] = true;
this.setState({
...state,
...data
});
}
)
);
}
render() {
return (
<Component {...this.state}
{...this.props}
/>
);
}
};
};
|
examples/with-global-stylesheet/pages/index.js | callumlocke/next.js | import React from 'react'
import stylesheet from 'styles/index.scss'
// or, if you work with plain css
// import stylesheet from 'styles/index.css'
export default () =>
<div>
<style dangerouslySetInnerHTML={{ __html: stylesheet }} />
<p>ciao</p>
</div>
|
app/javascript/mastodon/features/ui/components/upload_area.js | maa123/mastodon | import React from 'react';
import PropTypes from 'prop-types';
import Motion from '../../ui/util/optional_motion';
import spring from 'react-motion/lib/spring';
import { FormattedMessage } from 'react-intl';
export default class UploadArea extends React.PureComponent {
static propTypes = {
active: PropTypes.bool,
onClose: PropTypes.func,
};
handleKeyUp = (e) => {
const keyCode = e.keyCode;
if (this.props.active) {
switch(keyCode) {
case 27:
e.preventDefault();
e.stopPropagation();
this.props.onClose();
break;
}
}
}
componentDidMount () {
window.addEventListener('keyup', this.handleKeyUp, false);
}
componentWillUnmount () {
window.removeEventListener('keyup', this.handleKeyUp);
}
render () {
const { active } = this.props;
return (
<Motion defaultStyle={{ backgroundOpacity: 0, backgroundScale: 0.95 }} style={{ backgroundOpacity: spring(active ? 1 : 0, { stiffness: 150, damping: 15 }), backgroundScale: spring(active ? 1 : 0.95, { stiffness: 200, damping: 3 }) }}>
{({ backgroundOpacity, backgroundScale }) => (
<div className='upload-area' style={{ visibility: active ? 'visible' : 'hidden', opacity: backgroundOpacity }}>
<div className='upload-area__drop'>
<div className='upload-area__background' style={{ transform: `scale(${backgroundScale})` }} />
<div className='upload-area__content'><FormattedMessage id='upload_area.title' defaultMessage='Drag & drop to upload' /></div>
</div>
</div>
)}
</Motion>
);
}
}
|
js/components/settings/locations.js | stage88/react-weather | /**
* @flow
*/
'use strict';
import { connect } from 'react-redux';
import React, { Component } from 'react';
import {
StyleSheet,
View,
Text,
StatusBar,
ScrollView,
LayoutAnimation
} from 'react-native';
import Icon from 'react-native-vector-icons/Ionicons';
import Swipeout from '../../dependencies/swipeout';
import defaultStyles from './styles';
import { getAllLocations, deleteLocation } from '../../actions/location';
import type { Location } from '../../models/view';
import Section from './section';
import NavigationButtonRow from './navigationbuttonrow';
import AddLocation from './addlocation';
const renderForecastImage = require('../forecastimage')
type Props = {
navigator: any;
dispatch: any;
locations: Array<Location>;
count: number;
};
class Locations extends Component {
props: Props;
constructor(props: Props) {
super(props);
(this: any).close = this.close.bind(this);
}
componentWillMount() {
this.props.dispatch(getAllLocations());
}
render() {
var locations = this.props.locations.map((item) => {
var current = item.observation ? item.observation.current + '\u00B0' : "-";
var low = item.observation ? item.observation.low : "-";
var high = item.observation ? item.observation.high : "-";
var icon = item.observation ? renderForecastImage(item.observation.icon, 20, 20) : null;
return (
<Swipeout
key={item.openWeatherId}
autoClose={true}
right={[{text: 'Delete', backgroundColor: '#FF3B30', onPress: () => {
this.props.dispatch(deleteLocation(item.openWeatherId))
}}]}>
<View style={styles.locationRow}>
<View style={styles.locationLeft}>
<Text style={styles.locationNameText}>{ item.name }</Text>
<Text style={styles.locationCurrentText}>{ current }</Text>
</View>
<View style={styles.locationRight}>
<View style={{justifyContent: 'center', flexDirection: 'row'}}>
{ icon }
<Text style={styles.locationTextLow}>{ low }</Text>
<Text style={styles.locationTextHigh}>{ high }</Text>
</View>
</View>
</View>
</Swipeout>
);
});
return (
<View style={styles.container}>
<StatusBar barStyle='default' backgroundColor='#000' />
<ScrollView style={{flexDirection: 'column'}}>
{ locations }
<Section style={{marginTop: 36}}>
<NavigationButtonRow
text={'Add Location'}
component={AddLocation}
navigator={this.props.navigator} />
</Section>
</ScrollView>
</View>
);
}
close() {
this.props.navigator.close();
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'column',
backgroundColor: '#f8f8f8'
},
locationRow: {
flexDirection: 'row',
justifyContent: 'center',
alignItems: 'stretch',
backgroundColor: '#fff',
paddingLeft: 14,
paddingRight: 14,
height: 72,
borderColor: '#C8C7CC',
borderBottomWidth: 0.3
},
locationLeft: {
flex: 1,
justifyContent: 'center'
},
locationNameText: {
fontSize: 16
},
locationCurrentText: {
fontSize: 16,
color: '#B0B5BF'
},
locationRight: {
flex: 1,
justifyContent: 'flex-end',
alignItems: 'center',
flexDirection: 'row'
},
locationTextLow: {
textAlign: 'right',
marginLeft: 14,
width: 20,
color: '#B0B5BF',
fontSize: 16
},
locationTextHigh: {
textAlign: 'right',
marginLeft: 14,
width: 20,
fontSize: 16
}
});
function select(store: any, props: Props) {
return {
isRefreshing: store.weather.isRefreshing,
locations: store.location.data,
count: store.location.data.length,
...props
};
}
module.exports = connect(select)(Locations);
|
src/views/components/multimedia/MediaInputSelect.js | Domiii/project-empire | import map from 'lodash/map';
import filter from 'lodash/filter';
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import { dataBind } from 'dbdi/react';
import { getOptionalArgument, NOT_LOADED } from 'dbdi/util';
import FAIcon from 'src/views/components/util/FAIcon';
import LoadIndicator from 'src/views/components/util/LoadIndicator';
import filesize from 'filesize';
import {
Alert, Button, Jumbotron, Well, Panel
} from 'react-bootstrap';
import Flexbox from 'flexbox-react';
import Select from 'react-select';
//import { Promise } from 'firebase';
const validDeviceIdRegex = /[0-9A-Fa-f]{6}/g;
function selectByKind(kind, videoinput, audioinput) {
switch (kind) {
case 'videoinput':
return videoinput;
case 'audioinput':
return audioinput;
default:
return null;
}
}
function getStream(constraints) {
return window.navigator.mediaDevices.getUserMedia(constraints)
.then(mediaStream => {
return mediaStream;
})
.catch(err => {
throw new Error('Could not get stream - ' + (err.stack || err));
}); // always check for errors at the end.
}
function queryUnknownDevices(kind) {
return getDeviceList(kind).then(list => {
list = filter(list, info => !info.label);
const promises = map(list, info => {
const type = selectByKind(info.kind, 'video', 'audio');
const { deviceId } = info;
const constraints = {
[type]: {
deviceId
}
};
// open stream to request permission to show the label
return getStream(constraints);
});
// query all devices again, after they have all been resolved
return Promise.all(promises).then((streams) => {
// shutdown all streams again
streams.forEach(stream => stream && stream.getTracks().forEach(track => track.stop()));
return getDeviceList(kind);
});
});
}
function getDeviceList(kind) {
return window.navigator.mediaDevices.enumerateDevices().then(
list => filter(list, info =>
info.kind === kind &&
(!info.deviceId || validDeviceIdRegex.test(info.deviceId)))
);
}
function getMediaSelectOptions(kind) {
return getDeviceList(kind).then((list) => {
let hasUnkownDevices = false;
const options = map(list, info => ({
value: info.deviceId,
label: info.label || (hasUnkownDevices = true && (<span className="color-gray">
(裝置的名字被隱藏)
<FAIcon name="user-secret" /><FAIcon name="lock" />
</span>))
}));
return {
options,
hasUnkownDevices
};
});
}
@dataBind({
onSelectionChanged(option,
args,
{ set_videoDeviceId, set_audioDeviceId }
) {
const { kind } = args;
const onChange = getOptionalArgument(args, 'onChange');
const action = selectByKind(kind, set_videoDeviceId, set_audioDeviceId);
let deviceId = option && option.value;
action && action(deviceId);
onChange && onChange(deviceId);
}
})
export default class MediaInputSelect extends Component {
static propTypes = {
kind: PropTypes.string.isRequired,
onChange: PropTypes.func,
disabled: PropTypes.bool
};
constructor(...args) {
super(...args);
this.state = { options: NOT_LOADED };
this.dataBindMethods(
'refresh'
);
}
componentDidMount() {
this.refresh();
}
refresh = (
{ },
{ onSelectionChanged },
{ }
) => {
if (this.state.options) {
this.setState({ options: NOT_LOADED });
}
const {
kind
} = this.props;
// generate options from media device list
// see: https://developer.mozilla.org/en-US/docs/Web/API/MediaDeviceInfo
getMediaSelectOptions(kind).then(({
options,
hasUnkownDevices
}) => {
const wasLoaded = this.state.options !== NOT_LOADED;
const hasDevices = !!options.length;
const defaultDeviceInfo = options[0];
if (hasDevices) {
// add "no selection"
options.unshift({
value: null,
label: <span>clear <FAIcon color="red" name="times" /></span>
});
}
if (!wasLoaded && hasDevices) {
// select the first by default
onSelectionChanged(defaultDeviceInfo);
}
// update state
this.setState({
options,
hasUnkownDevices
});
});
}
clickQueryUnknownDevices = (evt) => {
const {
kind
} = this.props;
return queryUnknownDevices(kind).then(list => {
this.refresh();
});
}
clickRefresh = evt => {
this.refresh();
}
render(
{ kind },
{ onSelectionChanged, get_videoDeviceId, get_audioDeviceId },
{ }
) {
const { disabled } = this.props;
const { options, hasUnkownDevices } = this.state;
if (options === NOT_LOADED) {
return <LoadIndicator block message="" />;
}
const getter = selectByKind(kind, get_videoDeviceId, get_audioDeviceId);
if (!getter) {
return <Alert bsStyle="danger">[INTERNAL ERROR] invalid kind: {kind}</Alert>;
}
const placeholder = options.length ? <i>(no {kind} selected)</i> : <i>(no {kind} available)</i>;
return (<Flexbox className="full-width">
<Flexbox className="full-width">
<Select className="full-width"
value={getter()}
placeholder={placeholder}
options={options}
onChange={onSelectionChanged}
disabled={disabled}
/>
</Flexbox>
{hasUnkownDevices && (<Flexbox>
<Button bsStyle="success" onClick={this.clickQueryUnknownDevices}>
<FAIcon name="unlock" /> 顯示所有裝置的名字
</Button>
</Flexbox>) }
{!hasUnkownDevices && !disabled && (<Flexbox>
<Button bsStyle="primary" onClick={this.clickRefresh}>
<FAIcon name="refresh" />
</Button>
</Flexbox>)}
</Flexbox>);
}
} |
survivejs/app/components/Notes.js | leowmjw/playground-ui | import React from 'react';
import Note from './Note.jsx';
export default class Notes extends React.Component {
render() {
const notes = this.props.items;
return <ul className="notes">{notes.map(this.renderNote)}</ul>;
}
renderNote = (note) => {
return (
<li className="note" key={note.id}>
<Note
task={note.task}
onEdit={this.props.onEdit.bind(null, note.id)}
onDelete={this.props.onDelete.bind(null, note.id)}
/>
</li>
);
}
}
|
src/svg-icons/image/filter-vintage.js | mtsandeep/material-ui | import React from 'react';
import pure from 'recompose/pure';
import SvgIcon from '../../SvgIcon';
let ImageFilterVintage = (props) => (
<SvgIcon {...props}>
<path d="M18.7 12.4c-.28-.16-.57-.29-.86-.4.29-.11.58-.24.86-.4 1.92-1.11 2.99-3.12 3-5.19-1.79-1.03-4.07-1.11-6 0-.28.16-.54.35-.78.54.05-.31.08-.63.08-.95 0-2.22-1.21-4.15-3-5.19C10.21 1.85 9 3.78 9 6c0 .32.03.64.08.95-.24-.2-.5-.39-.78-.55-1.92-1.11-4.2-1.03-6 0 0 2.07 1.07 4.08 3 5.19.28.16.57.29.86.4-.29.11-.58.24-.86.4-1.92 1.11-2.99 3.12-3 5.19 1.79 1.03 4.07 1.11 6 0 .28-.16.54-.35.78-.54-.05.32-.08.64-.08.96 0 2.22 1.21 4.15 3 5.19 1.79-1.04 3-2.97 3-5.19 0-.32-.03-.64-.08-.95.24.2.5.38.78.54 1.92 1.11 4.2 1.03 6 0-.01-2.07-1.08-4.08-3-5.19zM12 16c-2.21 0-4-1.79-4-4s1.79-4 4-4 4 1.79 4 4-1.79 4-4 4z"/>
</SvgIcon>
);
ImageFilterVintage = pure(ImageFilterVintage);
ImageFilterVintage.displayName = 'ImageFilterVintage';
ImageFilterVintage.muiName = 'SvgIcon';
export default ImageFilterVintage;
|
client/src/components/roms/list/Container.js | DjLeChuck/recalbox-manager | import React, { Component } from 'react';
import PropTypes from 'prop-types';
import { translate } from 'react-i18next';
import { get } from '../../../api';
import { promisifyData, cancelPromises } from '../../../utils';
import RomsList from './List';
class RomsListContainer extends Component {
static propTypes = {
t: PropTypes.func.isRequired,
};
constructor(props) {
super(props);
this.state = {
loaded: false,
directoryListing: [],
esSystems: [],
};
}
async componentWillMount() {
const state = await promisifyData(
get('directoryListing'),
get('esSystems')
);
state.loaded = true;
this.setState(state);
}
componentWillUnmount() {
cancelPromises();
}
render() {
return (
<RomsList {...this.state} />
);
}
}
export default translate()(RomsListContainer);
|
app/containers/photos/UnsplashPage.js | foysalit/wallly-electron | // @flow
import React, { Component } from 'react';
import { Grid } from 'semantic-ui-react';
import List from '../../components/photos/List';
import Photos from '../../utils/photos';
const api = new Photos();
export default class UnsplashPage extends Component {
state = {photos: [], isLoading: true};
loadPhotos () {
this.setState({isLoading: true});
api.getPhotos().then(photos => {
this.setState({photos, isLoading: false});
});
};
componentDidMount() {
this.loadPhotos();
};
render() {
const { photos } = this.state;
return (
<Grid columns={2} style={{ padding: '2%' }}>
<Grid.Column width={10}>
<List
photos={photos}
loadMore={this.loadPhotos.bind(this)}
isLoading={this.state.isLoading}/>
</Grid.Column>
</Grid>
);
}
}
|
assets/javascripts/data_table.js | simonhildebrandt/redact | import $ from 'jquery'
import React from 'react'
import FloatingActionButton from 'material-ui/FloatingActionButton'
import {Table, TableBody, TableHeader, TableHeaderColumn, TableRow, TableRowColumn, TableFooter} from 'material-ui/Table'
import {BottomNavigation, BottomNavigationItem} from 'material-ui/BottomNavigation'
import ContentAdd from 'material-ui/svg-icons/content/add'
class DataTable extends React.Component {
constructor(props) {
super(props)
this.state = {
sort: this.primaryField().name,
page: 0,
data: null
}
}
componentDidMount() {
this.getData()
}
componentWillReceiveProps(nextProps) {
if (nextProps.model.name != this.props.model.name) {
this.setData(null)
}
}
componentDidUpdate() {
if (this.state.data == null) {
this.getData()
}
}
modelName() {
return this.props.model.name
}
pluralPath() {
return "/" + this.modelName()
}
getData() {
$.get(this.pluralPath())
.done((data) => {
this.setData(data)
})
}
setData(data) {
this.setState({data: data})
}
primaryField() {
return this.fields()[0] // TODO - ...or field.primary == true
}
addSortColumn(column) {
this.setState({sort: this.state.sort + [column]})
}
getTableHeight() {
return '100%'
}
fields() {
return this.props.model.fields
}
headers() {
return <TableHeader>
<TableRow>
{ this.fields().map((field) => { return this.header(field) }) }
</TableRow>
</TableHeader>
}
header(field) {
return <TableHeaderColumn key={field.name}>{field.label}</TableHeaderColumn>
}
rows() {
if (!this.state.data) { return }
return <TableBody>
{ this.state.data.map((data) => { return this.row(data) }) }
</TableBody>
}
row(record) {
return <TableRow key={record.id}>
{ this.fields().map((field) => { return this.column(field, record) } ) }
</TableRow>
}
column(field, record) {
return <TableRowColumn key={field.name}>{record[field.name]}</TableRowColumn>
}
render () {
return <div>
<Table height={this.getTableHeight()} multiSelectable={true} fixedHeader={true} fixedFooter={true}>
{this.headers()}
{ this.rows() }
<TableFooter>
<TableRow>
<TableRowColumn>ID</TableRowColumn>
<TableRowColumn>Name</TableRowColumn>
<TableRowColumn>Status</TableRowColumn>
</TableRow>
<TableRow>
<TableRowColumn colSpan="3" style={{textAlign: 'center'}}>
Super Footer
</TableRowColumn>
</TableRow>
</TableFooter>
</Table>
<FloatingActionButton style={ {
margin: 0,
top: 'auto',
right: 20,
bottom: 20,
left: 'auto',
position: 'fixed',
} }><ContentAdd />
</FloatingActionButton>
</div>
}
}
module.exports = DataTable
|
web/src/js/components/Widget/Widgets/WidgetPieceWrapper.js | gladly-team/tab | import React from 'react'
// import PropTypes from 'prop-types'
class WidgetPieceWrapper extends React.Component {
render() {
return <span>{this.props.children}</span>
}
}
WidgetPieceWrapper.propTypes = {}
WidgetPieceWrapper.defaultProps = {}
export default WidgetPieceWrapper
|
node_modules/semantic-ui-react/dist/es/elements/Step/StepTitle.js | mowbell/clickdelivery-fed-test | import _extends from 'babel-runtime/helpers/extends';
import _isNil from 'lodash/isNil';
import cx from 'classnames';
import PropTypes from 'prop-types';
import React from 'react';
import { customPropTypes, getElementType, getUnhandledProps, META } from '../../lib';
/**
* A step can contain a title.
*/
function StepTitle(props) {
var children = props.children,
className = props.className,
title = props.title;
var classes = cx('title', className);
var rest = getUnhandledProps(StepTitle, props);
var ElementType = getElementType(StepTitle, props);
return React.createElement(
ElementType,
_extends({}, rest, { className: classes }),
_isNil(children) ? title : children
);
}
StepTitle.handledProps = ['as', 'children', 'className', 'title'];
StepTitle._meta = {
name: 'StepTitle',
parent: 'Step',
type: META.TYPES.ELEMENT
};
process.env.NODE_ENV !== "production" ? StepTitle.propTypes = {
/** An element type to render as (string or function). */
as: customPropTypes.as,
/** Primary content. */
children: PropTypes.node,
/** Additional classes. */
className: PropTypes.string,
/** Shorthand for primary content. */
title: customPropTypes.contentShorthand
} : void 0;
export default StepTitle; |
app/javascript/mastodon/features/ui/components/navigation_panel.js | musashino205/mastodon | import React from 'react';
import { NavLink, withRouter } from 'react-router-dom';
import { FormattedMessage } from 'react-intl';
import Icon from 'mastodon/components/icon';
import { showTrends } from 'mastodon/initial_state';
import NotificationsCounterIcon from './notifications_counter_icon';
import FollowRequestsNavLink from './follow_requests_nav_link';
import ListPanel from './list_panel';
import TrendsContainer from 'mastodon/features/getting_started/containers/trends_container';
const NavigationPanel = () => (
<div className='navigation-panel'>
<NavLink className='column-link column-link--transparent' to='/home' data-preview-title-id='column.home' data-preview-icon='home' ><Icon className='column-link__icon' id='home' fixedWidth /><FormattedMessage id='tabs_bar.home' defaultMessage='Home' /></NavLink>
<NavLink className='column-link column-link--transparent' to='/notifications' data-preview-title-id='column.notifications' data-preview-icon='bell' ><NotificationsCounterIcon className='column-link__icon' /><FormattedMessage id='tabs_bar.notifications' defaultMessage='Notifications' /></NavLink>
<FollowRequestsNavLink />
<NavLink className='column-link column-link--transparent' to='/explore' data-preview-title-id='explore.title' data-preview-icon='hashtag'><Icon className='column-link__icon' id='hashtag' fixedWidth /><FormattedMessage id='explore.title' defaultMessage='Explore' /></NavLink>
<NavLink className='column-link column-link--transparent' to='/public/local' data-preview-title-id='column.community' data-preview-icon='users' ><Icon className='column-link__icon' id='users' fixedWidth /><FormattedMessage id='tabs_bar.local_timeline' defaultMessage='Local' /></NavLink>
<NavLink className='column-link column-link--transparent' exact to='/public' data-preview-title-id='column.public' data-preview-icon='globe' ><Icon className='column-link__icon' id='globe' fixedWidth /><FormattedMessage id='tabs_bar.federated_timeline' defaultMessage='Federated' /></NavLink>
<NavLink className='column-link column-link--transparent' to='/conversations'><Icon className='column-link__icon' id='envelope' fixedWidth /><FormattedMessage id='navigation_bar.direct' defaultMessage='Direct messages' /></NavLink>
<NavLink className='column-link column-link--transparent' to='/favourites'><Icon className='column-link__icon' id='star' fixedWidth /><FormattedMessage id='navigation_bar.favourites' defaultMessage='Favourites' /></NavLink>
<NavLink className='column-link column-link--transparent' to='/bookmarks'><Icon className='column-link__icon' id='bookmark' fixedWidth /><FormattedMessage id='navigation_bar.bookmarks' defaultMessage='Bookmarks' /></NavLink>
<NavLink className='column-link column-link--transparent' to='/lists'><Icon className='column-link__icon' id='list-ul' fixedWidth /><FormattedMessage id='navigation_bar.lists' defaultMessage='Lists' /></NavLink>
<ListPanel />
<hr />
<a className='column-link column-link--transparent' href='/settings/preferences'><Icon className='column-link__icon' id='cog' fixedWidth /><FormattedMessage id='navigation_bar.preferences' defaultMessage='Preferences' /></a>
<a className='column-link column-link--transparent' href='/relationships'><Icon className='column-link__icon' id='users' fixedWidth /><FormattedMessage id='navigation_bar.follows_and_followers' defaultMessage='Follows and followers' /></a>
{showTrends && <div className='flex-spacer' />}
{showTrends && <TrendsContainer />}
</div>
);
export default withRouter(NavigationPanel);
|
node_modules/react-bootstrap/es/ModalDialog.js | vietvd88/developer-crawler | import _extends from 'babel-runtime/helpers/extends';
import _objectWithoutProperties from 'babel-runtime/helpers/objectWithoutProperties';
import _classCallCheck from 'babel-runtime/helpers/classCallCheck';
import _possibleConstructorReturn from 'babel-runtime/helpers/possibleConstructorReturn';
import _inherits from 'babel-runtime/helpers/inherits';
import classNames from 'classnames';
import React from 'react';
import { bsClass, bsSizes, getClassSet, prefix, splitBsProps } from './utils/bootstrapUtils';
import { Size } from './utils/StyleConfig';
var propTypes = {
/**
* A css class to apply to the Modal dialog DOM node.
*/
dialogClassName: React.PropTypes.string
};
var ModalDialog = function (_React$Component) {
_inherits(ModalDialog, _React$Component);
function ModalDialog() {
_classCallCheck(this, ModalDialog);
return _possibleConstructorReturn(this, _React$Component.apply(this, arguments));
}
ModalDialog.prototype.render = function render() {
var _extends2;
var _props = this.props,
dialogClassName = _props.dialogClassName,
className = _props.className,
style = _props.style,
children = _props.children,
props = _objectWithoutProperties(_props, ['dialogClassName', 'className', 'style', 'children']);
var _splitBsProps = splitBsProps(props),
bsProps = _splitBsProps[0],
elementProps = _splitBsProps[1];
var bsClassName = prefix(bsProps);
var modalStyle = _extends({ display: 'block' }, style);
var dialogClasses = _extends({}, getClassSet(bsProps), (_extends2 = {}, _extends2[bsClassName] = false, _extends2[prefix(bsProps, 'dialog')] = true, _extends2));
return React.createElement(
'div',
_extends({}, elementProps, {
tabIndex: '-1',
role: 'dialog',
style: modalStyle,
className: classNames(className, bsClassName)
}),
React.createElement(
'div',
{ className: classNames(dialogClassName, dialogClasses) },
React.createElement(
'div',
{ className: prefix(bsProps, 'content'), role: 'document' },
children
)
)
);
};
return ModalDialog;
}(React.Component);
ModalDialog.propTypes = propTypes;
export default bsClass('modal', bsSizes([Size.LARGE, Size.SMALL], ModalDialog)); |
app/components/ProjectCard.js | georgeF105/georgeF105.github.io | import React from 'react'
export default (props) => {
const project = props.project
return (
<div className='project-card card'>
<h3>Project {project.name}</h3>
<div className='card-links'>
{project.url ? <a href={project.url} className='fa fa-desktop' /> : null}
{project.github_url ? <a href={project.github_url} className='fa fa-github' /> : null}
</div>
<p>{project.description}</p>
</div>
)
}
|
1l_instastack/src/index.js | yevheniyc/Projects | import 'babel-polyfill'; // for redux-saga
import React from 'react';
import ReactDOM from 'react-dom';
import {
Router,
Route,
hashHistory
} from 'react-router';
import {
createStore,
applyMiddleware,
compose
} from 'redux';
import reducer from './reducer';
import { Provider } from 'react-redux';
import createSagaMiddleware from 'redux-saga';
import rootSaga from './sagas';
// our components
import Layout from './components/layout';
import { HomeContainer } from './components/home';
import { DetailContainer } from './components/detail';
import { AddContainer } from './components/add';
// app css
import '../dist/css/style.css';
// Filestack API requires to set a key
filepicker.setKey("YOUR_API_KEY");
const sagaMiddleware = createSagaMiddleware();
const store = createStore(
reducer,
compose(
applyMiddleware(sagaMiddleware),
window.devToolsExtension ? window.devToolsExtension() : f => f // connect to redux devtools
)
);
sagaMiddleware.run(rootSaga);
// the 3 paths of the app
const routes = <Route component={Layout}>
<Route path="/" component={HomeContainer} />
<Route path="/detail/:id" component={DetailContainer} />
<Route path="/add" component={AddContainer} />
</Route>;
// add provider as first component and connect the store to it
ReactDOM.render(
<Provider store={store}>
<Router history={hashHistory}>{routes}</Router>
</Provider>,
document.getElementById('app')
);
|
Week02-React-Jest-Unit-Tests/client/src/index.js | bmeansjd/ISIT320_means2017 | import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import registerServiceWorker from './registerServiceWorker';
ReactDOM.render(<App />, document.getElementById('root'));
registerServiceWorker();
|
src/index.js | amsardesai/react-fontawesome | import React from 'react'
/**
* A React component for the font-awesome icon library.
*
*
* @param {Boolean} [border=false] Whether or not to show a border radius
* @param {String} [className] An extra set of CSS classes to add to the component
* @param {Boolean} [fixedWidth=false] Make buttons fixed width
* @param {String} [flip=false] Flip the icon's orientation.
* @param {Boolean} [inverse=false]Inverse the icon's color
* @param {String} name Name of the icon to use
* @param {Boolean} [pulse=false] Rotate icon with 8 steps (rather than smoothly)
* @param {Number} [rotate] The degress to rotate the icon by
* @param {String} [size] The icon scaling size
* @param {Boolean} [spin=false] Spin the icon
* @param {String} [stack] Stack an icon on top of another
* @module FontAwesome
* @type {ReactClass}
*/
export default React.createClass({
displayName: 'FontAwesome',
propTypes: {
border: React.PropTypes.bool,
className: React.PropTypes.string,
fixedWidth: React.PropTypes.bool,
flip: React.PropTypes.oneOf([ 'horizontal', 'vertical' ]),
inverse: React.PropTypes.bool,
name: React.PropTypes.string.isRequired,
pulse: React.PropTypes.bool,
rotate: React.PropTypes.oneOf([ 90, 180, 270 ]),
size: React.PropTypes.oneOf([ 'lg', '2x', '3x', '4x', '5x' ]),
spin: React.PropTypes.bool,
stack: React.PropTypes.oneOf([ '1x', '2x' ]),
},
render() {
let className = 'fa fa-' + this.props.name
if (this.props.size) {
className += ' fa-' + this.props.size
}
if (this.props.spin) {
className += ' fa-spin'
}
if (this.props.pulse) {
className += ' fa-pulse'
}
if (this.props.border) {
className += ' fa-border'
}
if (this.props.fixedWidth) {
className += ' fa-fw'
}
if (this.props.inverse) {
className += ' fa-inverse'
}
if (this.props.flip) {
className += ' fa-flip-' + this.props.flip
}
if (this.props.rotate) {
className += ' fa-rotate-' + this.props.rotate
}
if (this.props.stack) {
className += ' fa-stack-' + this.props.stack
}
if (this.props.className) {
className += ' ' + this.props.className
}
return (
<span
{...this.props}
className={className}
/>
)
},
})
|
src/index.js | LeoAJ/react-facebook-friends | import 'babel-polyfill';
import React from 'react';
import { render } from 'react-dom';
import App from './components/App';
// import CSS
import '../vendor/css/gh-fork-ribbon.css';
import '../vendor/css/font-awesome.css';
import '../vendor/css/base.css';
import '../vendor/css/buttons.css';
render(
<App />,
document.querySelector('#root')
);
|
dist/elements/Checkbox/index.js | codespec/react-toolset | function _defineProperty(obj, key, value) { if (key in obj) { Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true }); } else { obj[key] = value; } return obj; }
function _objectWithoutProperties(obj, keys) { var target = {}; for (var i in obj) { if (keys.indexOf(i) >= 0) continue; if (!Object.prototype.hasOwnProperty.call(obj, i)) continue; target[i] = obj[i]; } return target; }
import React from 'react';
import PropTypes from 'prop-types';
import cx from 'classnames/bind';
import Icon from '../../icons/Icon';
import style from './style.css';
var classNames = cx.bind(style);
var Checkbox = function Checkbox(_ref) {
var id = _ref.id,
size = _ref.size,
type = _ref.type,
isChecked = _ref.isChecked,
isDisabled = _ref.isDisabled,
className = _ref.className,
onChange = _ref.onChange,
props = _objectWithoutProperties(_ref, ['id', 'size', 'type', 'isChecked', 'isDisabled', 'className', 'onChange']);
var labelClass = classNames('Checkbox',
/* eslint-disable quote-props */
{ 'Checkbox--disabled': isDisabled, 'Checkbox--checked': isChecked },
/* eslint-enable quote-props */
className.root);
var iconSizeMap = {
small: 14,
medium: 20,
large: 32,
xlarge: 45
};
var iconProps = {
name: 'GoCheck',
type: 'githubOcticons',
size: iconSizeMap[size]
};
if (type === 'dark') iconProps.color = '#fff';
return React.createElement(
'label',
{ htmlFor: id, className: labelClass },
React.createElement('input', {
className: classNames('Checkbox__native'),
type: 'checkbox',
id: id,
checked: isChecked,
onChange: onChange,
disabled: isDisabled
}),
React.createElement(
'span',
{ className: classNames('Checkbox__box', _defineProperty({
'Checkbox__box--checked': isChecked && type !== 'dark',
'Checkbox__box--checked--disabled': isChecked && isDisabled,
'Checkbox__box--checked-dark': isChecked && type === 'dark',
'Checkbox__box--disabled': isDisabled
}, 'Checkbox__box--' + size, true), className.box) },
isChecked && React.createElement(
'span',
{ className: classNames('Checkbox__checkmark', _defineProperty({}, 'Checkbox__checkmark--' + size, true)) },
React.createElement(Icon, iconProps)
)
),
React.createElement(
'span',
{ className: classNames('Checkbox__label', _defineProperty({
'Checkbox__label--disabled': isDisabled
}, 'Checkbox__label--' + size, true), className.labelText) },
props.children
)
);
};
Checkbox.propTypes = {
/** Value for `htmlFor` of label and `id` of input attribute for accessibility */
id: PropTypes.string.isRequired,
size: PropTypes.oneOf(['small', 'medium', 'large', 'xlarge']),
/** Type of checkbox style */
type: PropTypes.oneOf(['light', 'dark']),
/** Checked status of checkbox */
isChecked: PropTypes.bool,
/** Disabled status of checkbox */
isDisabled: PropTypes.bool,
/** Customizing style for checkbox element */
className: PropTypes.shape({
/** Additional class on root element */
root: PropTypes.string,
/** Additional class on square box element */
box: PropTypes.string,
/** Additional class on checkbox label text element */
labelText: PropTypes.string
}),
/** Input change event handler */
onChange: PropTypes.func,
children: PropTypes.node
};
Checkbox.defaultProps = {
size: 'small',
type: 'light',
isChecked: false,
isDisabled: false,
className: {}
};
export default Checkbox; |
lib/index.js | rebem/rebem | import React from 'react';
import { stringify, validate } from 'rebem-classname';
let buildClassName = stringify;
// validation
// istanbul ignore next
if (process.env.NODE_ENV !== 'production') {
buildClassName = props => stringify(validate(props));
}
function BEM(props, ...children) {
const { tag, block, elem, mods, mix, className, ...restProps } = props;
const finalClassName = buildClassName({ block, elem, mods, mix, className });
const finalProps = finalClassName ? { ...restProps, className: finalClassName } : restProps;
return React.createElement(tag || 'div', finalProps, ...children);
}
function blockFactory(block) {
return function (props, ...children) {
return BEM({ ...props, block }, ...children);
};
}
export { BEM, blockFactory };
|
src/components/common/svg-icons/content/undo.js | abzfarah/Pearson.NAPLAN.GnomeH | import React from 'react';
import pure from 'recompose/pure';
import SvgIcon from '../../SvgIcon';
let ContentUndo = (props) => (
<SvgIcon {...props}>
<path d="M12.5 8c-2.65 0-5.05.99-6.9 2.6L2 7v9h9l-3.62-3.62c1.39-1.16 3.16-1.88 5.12-1.88 3.54 0 6.55 2.31 7.6 5.5l2.37-.78C21.08 11.03 17.15 8 12.5 8z"/>
</SvgIcon>
);
ContentUndo = pure(ContentUndo);
ContentUndo.displayName = 'ContentUndo';
ContentUndo.muiName = 'SvgIcon';
export default ContentUndo;
|
src/icons/KeyboardArrowLeftIcon.js | kiloe/ui | import React from 'react';
import Icon from '../Icon';
export default class KeyboardArrowLeftIcon extends Icon {
getSVG(){return <svg xmlns="http://www.w3.org/2000/svg" width="48" height="48" viewBox="0 0 48 48"><path d="M30.83 32.67l-9.17-9.17 9.17-9.17L28 11.5l-12 12 12 12z"/></svg>;}
}; |
node_modules/react-bootstrap/es/InputGroupButton.js | lucketta/got-quote-generator | import _extends from 'babel-runtime/helpers/extends';
import _objectWithoutProperties from 'babel-runtime/helpers/objectWithoutProperties';
import _classCallCheck from 'babel-runtime/helpers/classCallCheck';
import _possibleConstructorReturn from 'babel-runtime/helpers/possibleConstructorReturn';
import _inherits from 'babel-runtime/helpers/inherits';
import classNames from 'classnames';
import React from 'react';
import { bsClass, getClassSet, splitBsProps } from './utils/bootstrapUtils';
var InputGroupButton = function (_React$Component) {
_inherits(InputGroupButton, _React$Component);
function InputGroupButton() {
_classCallCheck(this, InputGroupButton);
return _possibleConstructorReturn(this, _React$Component.apply(this, arguments));
}
InputGroupButton.prototype.render = function render() {
var _props = this.props,
className = _props.className,
props = _objectWithoutProperties(_props, ['className']);
var _splitBsProps = splitBsProps(props),
bsProps = _splitBsProps[0],
elementProps = _splitBsProps[1];
var classes = getClassSet(bsProps);
return React.createElement('span', _extends({}, elementProps, {
className: classNames(className, classes)
}));
};
return InputGroupButton;
}(React.Component);
export default bsClass('input-group-btn', InputGroupButton); |
step9-redux/node_modules/react-router/modules/IndexLink.js | jintoppy/react-training | import React from 'react'
import Link from './Link'
/**
* An <IndexLink> is used to link to an <IndexRoute>.
*/
const IndexLink = React.createClass({
render() {
return <Link {...this.props} onlyActiveOnIndex={true} />
}
})
export default IndexLink
|
src/components/Label/Label.example.js | 6congyao/CGB-Dashboard | /*
* Copyright (c) 2016-present, Parse, LLC
* All rights reserved.
*
* This source code is licensed under the license found in the LICENSE file in
* the root directory of this source tree.
*/
import React from 'react';
import Field from 'components/Field/Field.react';
import Label from 'components/Label/Label.react';
export const component = Label;
export const demos = [
{
render: () => (
<Field
label={<Label text='This is my text.' description='This is my description.' />}
input={null} />
)
}
];
|
frontend-web-v2/src/components/LightToggle.js | ClubCedille/jardiniot | import React from 'react';
import Slider from 'rc-slider';
import API from '../Api';
export default class LightToggle extends React.Component {
constructor(props) {
super(props);
// set state..
this.state = {
...props,
}
}
render() {
return (
<div className="color">
<div className="name">
{this.state.light.name}
</div>
<div>
<Slider
max={255}
value={parseInt(this.state.light.value)}
onChange={this.handleLightChange}
onAfterChange={this.handleLightAfterChange}
className="slider"
trackStyle={{
backgroundColor: this.state.light.name.toLowerCase(),
}}
handleStyle={{
border: `2px solid ${this.state.light.name.toLowerCase()}`
}}
/>
</div>
</div>
)
}
handleLightAfterChange = async (value) => {
await this.props.changeValue(value);
}
handleLightChange = (value) => {
const { light } = this.state
this.setState({ light: { ...light, value } });
}
}
|
packages/slate-html-serializer/test/serialize/block-nested.js | 6174/slate |
/** @jsx h */
import React from 'react'
import h from '../helpers/h'
export const rules = [
{
serialize(obj, children) {
if (obj.kind != 'block') return
switch (obj.type) {
case 'paragraph': return React.createElement('p', {}, children)
case 'quote': return React.createElement('blockquote', {}, children)
}
}
}
]
export const input = (
<state>
<document>
<quote>
<paragraph>
one
</paragraph>
</quote>
</document>
</state>
)
export const output = `
<blockquote><p>one</p></blockquote>
`.trim()
|
step-capstone/src/components/TravelObjectForms/FormPopover.js | googleinterns/step98-2020 | import React from 'react';
import {Popover} from '@material-ui/core';
import ItemForm from './ItemForm'
export default function FormPopver(props) {
return (
<div>
<Popover
open={props.open}
anchorReference={props.anchorReference}
anchorPosition={props.anchorPosition}
anchorEl={props.anchorEl}
onClose={props.onClose}
anchorOrigin={props.anchorOrigin}
transformOrigin={props.transformOrigin}
>
<ItemForm
onClose={props.onClose}
onAddItem={props.onAddItem}
onEditItem={props.onEditItem}
onRemoveItem={props.onRemoveItem}
data={props.data}
isNewItem={props.isNewItem}
startDate={props.startDate}
travelObjects={props.travelObjects}
isFromSuggestions={props.isFromSuggestions}
/>
</Popover>
</div>
)
} |
src/components/AppSettingsMenu/ThemeControls/ThemeSettings.js | Charlie9830/pounder | import React, { Component } from 'react';
import { withTheme } from '@material-ui/core';
import { GetMuiColorArray, GetColor } from '../../../utilities/MuiColors';
import { connect } from 'react-redux';
import {
createNewMuiThemeAsync, updateMuiThemeAsync, selectMuiTheme, renameMuiThemeAsync,
removeMuiThemeAsync,
persistMuiThemeSelection
} from 'handball-libs/libs/pounder-redux/action-creators';
import TransitionList from '../../TransitionList/TransitionList';
import ListItemTransition from '../../TransitionList/ListItemTransition';
import SwipeableListItem from '../../SwipeableListItem/SwipeableListItem';
import DeleteIcon from '@material-ui/icons/Delete';
import ThemeEditor from './ThemeEditor';
import ThemeListItem from './ThemeListItem';
class ThemeSettings extends Component {
constructor(props) {
super(props);
// Method Bindings.
this.extractSelectedMuiTheme = this.extractSelectedMuiTheme.bind(this);
this.getThemesJSX = this.getThemesJSX.bind(this);
this.randomize = this.randomize.bind(this);
}
componentWillUnmount() {
this.props.dispatch(persistMuiThemeSelection());
}
render() {
let muiColors = GetMuiColorArray();
let muiThemeEntity = this.extractSelectedMuiTheme();
if (muiThemeEntity === undefined) {
return null;
}
return (
<React.Fragment>
<TransitionList>
{this.getThemesJSX()}
</TransitionList>
<ThemeEditor
muiTheme={muiThemeEntity.theme}
onCreate={() => { this.props.dispatch(createNewMuiThemeAsync()) }}
isOpen={muiThemeEntity.isInbuilt === false}
muiColors={muiColors}
onThemeChange={(newTheme) => { this.props.dispatch(updateMuiThemeAsync(this.props.selectedMuiThemeId, newTheme)) }} />
{/*
<Button variant="contained" onClick={() => {this.randomize()}} > I'm Feeling Lucky </Button>
*/}
</React.Fragment>
);
}
getThemesJSX() {
if (this.props.muiThemes === undefined) {
return null;
}
let muiThemes = [...this.props.muiThemes];
muiThemes.sort((a, b) => {
return b.isInbuilt - a.isInbuilt;
})
let jsx = muiThemes.map( item => {
return (
<ListItemTransition
key={item.id}>
<ThemeListItem
name={item.name}
isSelected={item.id === this.props.selectedMuiThemeId}
primaryColor={GetColor(item.theme.palette.primaryColor.id, item.theme.palette.primaryColor.shadeIndex)}
secondaryColor={GetColor(item.theme.palette.secondaryColor.id, item.theme.palette.secondaryColor.shadeIndex)}
backgroundColor={GetColor(item.theme.palette.backgroundColor.id, item.theme.palette.backgroundColor.shadeIndex)}
onClick={() => { this.props.dispatch(selectMuiTheme(item.id)) }}
onDoubleClick={() => { this.props.dispatch(renameMuiThemeAsync(item.id)) }}
onDeleteButtonClick={() => { this.props.dispatch(removeMuiThemeAsync(item.id))}}
canDelete={item.isInbuilt === false}/>
</ListItemTransition>
)
})
return jsx;
}
randomize() {
let getRandomInt = (min, max) => {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min + 1)) + min;
}
let newTheme = {...this.extractSelectedMuiTheme()};
let colors = GetMuiColorArray();
let colorMax = colors.length - 1;
let shadeMax = 13;
newTheme.theme.palette.primaryColor = {
id: colors[getRandomInt(0, colorMax)].id,
shadeIndex: getRandomInt(0, shadeMax)
}
newTheme.theme.palette.secondaryColor = {
id: colors[getRandomInt(0, colorMax)].id,
shadeIndex: getRandomInt(0, shadeMax)
}
newTheme.theme.palette.backgroundColor = {
id: colors[getRandomInt(0, colorMax)].id,
shadeIndex: getRandomInt(0, shadeMax)
}
this.props.dispatch(updateMuiThemeAsync(newTheme.id, newTheme.theme));
}
extractSelectedMuiTheme() {
return this.props.muiThemes.find( item => {
return item.id === this.props.selectedMuiThemeId;
})
}
}
let mapStateToProps = state => {
return {
muiThemes: state.muiThemes,
selectedMuiThemeId: state.selectedMuiThemeId,
}
}
let VisibleThemeSettings = connect(mapStateToProps)(withTheme()(ThemeSettings));
export default VisibleThemeSettings; |
geonode/monitoring/frontend/src/components/cels/response-time/index.js | ppasq/geonode | import React from 'react';
import PropTypes from 'prop-types';
import { LineChart, Line, XAxis, YAxis, CartesianGrid, Tooltip, Legend } from 'recharts';
import styles from './styles';
class ResponseTime extends React.Component {
static propTypes = {
average: PropTypes.number.isRequired,
data: PropTypes.array.isRequired,
max: PropTypes.number.isRequired,
}
render() {
let latestResponse = 0;
for (let i = this.props.data.length - 1; i >= 0; --i) {
const response = this.props.data[i].time;
if (response !== 0) {
latestResponse = response;
break;
}
}
return (
<div style={styles.content}>
<h4>Response Time</h4>
Last Response Time: {latestResponse} ms<br />
Max Response Time: {this.props.max} ms<br />
Average Response Time: {this.props.average} ms<br />
<LineChart
width={500}
height={300}
data={this.props.data}
margin={{ top: 5, right: 30, left: 20, bottom: 5 }}
>
<XAxis dataKey="name" />
<YAxis />
<CartesianGrid strokeDasharray="3 3" />
<Tooltip />
<Legend />
<Line type="monotone" dataKey="time" stroke="#8884d8" activeDot={{ r: 8 }} />
</LineChart>
</div>
);
}
}
export default ResponseTime;
|
src/components/Button.android.js | madox2/fortune-app | import React from 'react'
import {View, TouchableNativeFeedback, Text, StyleSheet} from 'react-native'
export const Button = ({children, onPress, style}) => (
<TouchableNativeFeedback
onPress={onPress}
background={TouchableNativeFeedback.SelectableBackground()}>
<View style={[styles.button, style]}>
<Text style={styles.label}>{children}</Text>
</View>
</TouchableNativeFeedback>
)
const styles = StyleSheet.create({
button: {
padding: 14,
paddingRight: 20,
paddingLeft: 20,
flex: 1,
alignItems: 'center',
borderWidth: 1,
borderColor: 'silver',
},
label: {
fontSize: 18,
},
})
|
src/index.js | gor181/react-redux-mini-gallery | require('!style!css!./styles/index.css');
require('!style!css!muicss/lib/css/mui.css');
import React from 'react';
import { render } from 'react-dom';
import { Provider } from 'react-redux';
import MuiThemeProvider from 'material-ui/styles/MuiThemeProvider';
import injectTapEventPlugin from 'react-tap-event-plugin';
import store from './store';
import Layout from './layout';
injectTapEventPlugin();
render(
<MuiThemeProvider>
<Provider store={store}>
<Layout />
</Provider>
</MuiThemeProvider>,
document.getElementById('root')
);
|
packages/flow-runtime-docs/src/components/docs/PrimitiveTypesPage.js | codemix/flow-runtime | /* @flow */
import React, { Component } from 'react';
import {observer} from 'mobx-react';
import Example from '../Example';
const nullCode = `
import t from 'flow-runtime';
console.log(t.null().assert(null)); // ok
t.null().assert(undefined); // throws
t.null().assert(false); // throws
`.trim();
const voidCode = `
import t from 'flow-runtime';
console.log(t.void().assert(undefined)); // ok
t.void().assert(null); // throws
t.void().assert(false); // throws
`.trim();
const numberCode = `
import t from 'flow-runtime';
console.log(t.number().assert(123)); // ok
t.number().assert("456"); // throws
t.number().assert(null); // throws
`.trim();
const numberExactCode = `
import t from 'flow-runtime';
console.log(t.number(123).assert(123)); // ok
t.number(123).assert(123.456); // throws
t.number(123).assert(456); // throws
t.number(123).assert("123"); // throws
`.trim();
const booleanCode = `
import t from 'flow-runtime';
console.log(t.boolean().assert(true)); // ok
console.log(t.boolean().assert(false)); // ok
t.boolean().assert("456"); // throws
t.boolean().assert(null); // throws
`.trim();
const booleanExactCode = `
import t from 'flow-runtime';
console.log(t.boolean(true).assert(true)); // ok
t.boolean(true).assert(false); // throws
t.boolean(true).assert(456); // throws
t.boolean(true).assert("true"); // throws
`.trim();
const stringCode = `
import t from 'flow-runtime';
console.log(t.string().assert("foo")); // ok
t.string().assert(123); // throws
t.string().assert(null); // throws
`.trim();
const stringExactCode = `
import t from 'flow-runtime';
console.log(t.string("foo").assert("foo")); // ok
t.string("foo").assert("foobar"); // throws
t.string("foo").assert(false); // throws
`.trim();
const symbolCode = `
import t from 'flow-runtime';
console.log(t.symbol().assert(Symbol.for('test'))); // ok
t.symbol().assert("foobar"); // throws
t.symbol().assert(null); // throws
`.trim();
const symbolExactCode = `
import t from 'flow-runtime';
console.log(t.symbol(Symbol.for('test')).assert(Symbol.for('test'))); // ok
t.symbol(Symbol.for('test')).assert(Symbol('nope')); // throws
t.symbol(Symbol.for('test')).assert(456); // throws
t.symbol(Symbol.for('test')).assert("123"); // throws
`.trim();
const anyCode = `
import t from 'flow-runtime';
console.log(t.any().assert("foobar")); // ok
console.log(t.any().assert(null)); // ok
`.trim();
const mixedCode = `
import t from 'flow-runtime';
console.log(t.mixed().assert("foobar")); // ok
console.log(t.mixed().assert(null)); // ok
`.trim();
const nullableCode = `
import t from 'flow-runtime';
console.log(t.nullable(t.string()).assert("foobar")); // ok
console.log(t.nullable(t.string()).assert()); // ok
console.log(t.nullable(t.string()).assert(undefined)); // ok
console.log(t.nullable(t.string()).assert(null)); // ok
console.log(t.nullable(t.string()).assert(123)); // throws
`.trim();
@observer
export default class PrimitiveTypesPage extends Component {
render() {
return (
<div>
<header className="jumbotron jumbotron-fluid text-xs-center">
<h1>Primitive Types</h1>
</header>
<div className="container">
<h4>t.null()</h4>
<p><code>t.null()</code> matches exactly <code>null</code> and nothing else:</p>
<Example code={nullCode} hideOutput inline/>
<hr />
<h4>t.void()</h4>
<p><code>t.void()</code> matches exactly <code>undefined</code> and nothing else:</p>
<Example code={voidCode} hideOutput inline/>
<hr />
<h4>t.number()</h4>
<p><code>t.number()</code> matches any kind of number.</p>
<Example code={numberCode} hideOutput inline/>
<hr />
<h4>t.number(123)</h4>
<p><code>t.number(123)</code> matches a number with the exact value of <code>123</code>.</p>
<Example code={numberExactCode} hideOutput inline/>
<hr />
<h4>t.boolean()</h4>
<p><code>t.boolean()</code> matches any kind of boolean.</p>
<Example code={booleanCode} hideOutput inline/>
<hr />
<h4>t.boolean(true)</h4>
<p><code>t.boolean(true)</code> matches a boolean with the exact value of <code>true</code>.</p>
<Example code={booleanExactCode} hideOutput inline/>
<hr />
<h4>t.string()</h4>
<p><code>t.string()</code> matches any kind of string.</p>
<Example code={stringCode} hideOutput inline/>
<hr />
<h4>t.string("foo")</h4>
<p><code>t.string("foo")</code> matches a string with the exact value of <code>"foo"</code>.</p>
<Example code={stringExactCode} hideOutput inline/>
<hr />
<h4>t.symbol()</h4>
<p><code>t.symbol()</code> matches any kind of symbol.</p>
<Example code={symbolCode} hideOutput inline/>
<hr />
<h4>t.symbol(Symbol("abc"))</h4>
<p><code>t.symbol(Symbol("abc"))</code> matches an exact symbol.</p>
<Example code={symbolExactCode} hideOutput inline/>
<hr />
<h4>t.any()</h4>
<p><code>t.any()</code> matches any kind of value.</p>
<Example code={anyCode} hideOutput inline/>
<hr />
<h4>t.mixed()</h4>
<p><code>t.mixed()</code> matches any kind of value.</p>
<Example code={mixedCode} hideOutput inline/>
<hr />
<h4>t.nullable(type)</h4>
<p><code>t.nullable(type)</code> matches a <code>type</code> but also accepts <code>null</code> or <code>undefined</code>.</p>
<Example code={nullableCode} hideOutput inline/>
</div>
</div>
);
}
}
|
src/components/about.js | antholord/poe-livesearch | /**
* Created by Anthony Lord on 2017-05-02.
*/
import React, { Component } from 'react';
import Header from "./header";
class About extends Component{
render(){
return (
<div>
<Header league={""} onLeagueChange={null}/>
<div className="container main">
<h3><p>This website is a small project inspired by github.com/ccbrown who made a simple indexer that listens to the PoE item river. The main issue was that any user using the app has to download the whole river (over 1mb/s of data)</p><br />
<p>Instead, I made a backend api that listens to the river. The clients can thus subscribe to the API with a search request and only recieve the items that they searched for.</p><br />
<p>Since there's no indexing, items should show up on here faster than other sites that process and index items before making them available.</p><br />
<p>All of the code is open source so I recommend those interested to contribute. I used this project to learn new techs and I recommend other enthusiasts to do the same.</p><br />
<p>I plan to add support to search for mods but not much else.</p>
</h3>
<h2>contact : antholord@hotmail.com</h2>
<h2>Source code : <a href="https://github.com/antholord/poe-livesearch">Front end</a> | <a href="https://github.com/antholord/poe-livesearch-api">Back end</a></h2>
</div>
</div>
);
}
}
export default About; |
node_modules/semantic-ui-react/src/views/Comment/CommentContent.js | mowbell/clickdelivery-fed-test | import cx from 'classnames'
import PropTypes from 'prop-types'
import React from 'react'
import {
customPropTypes,
getElementType,
getUnhandledProps,
META,
} from '../../lib'
/**
* A comment can contain content.
*/
function CommentContent(props) {
const { className, children } = props
const classes = cx(className, 'content')
const rest = getUnhandledProps(CommentContent, props)
const ElementType = getElementType(CommentContent, props)
return <ElementType {...rest} className={classes}>{children}</ElementType>
}
CommentContent._meta = {
name: 'CommentContent',
parent: 'Comment',
type: META.TYPES.VIEW,
}
CommentContent.propTypes = {
/** An element type to render as (string or function). */
as: customPropTypes.as,
/** Primary content. */
children: PropTypes.node,
/** Additional classes. */
className: PropTypes.string,
}
export default CommentContent
|
src/app/containers/Statistics/components/LargeModal.js | MarcusKolman/www | import React, { Component } from 'react';
import { Modal, Button } from 'react-bootstrap';
//import LinkDetail from './link_detail';
class LargeModal extends Component {
render() {
return (
<Modal {...this.props} bsSize="large" aria-labelledby="contained-modal-title-lg">
<Modal.Header closeButton>
<Modal.Title id="contained-modal-title-lg">Modal heading</Modal.Title>
</Modal.Header>
<Modal.Body>
<h4>Wrapped Text</h4>
<p>Cras mattis consectetur purus sit amet fermentum. Cras justo odio, dapibus ac facilisis in, egestas eget quam. Morbi leo risus, porta ac consectetur ac, vestibulum at eros.</p>
<p>Praesent commodo cursus magna, vel scelerisque nisl consectetur et. Vivamus sagittis lacus vel augue laoreet rutrum faucibus dolor auctor.</p>
<p>Aenean lacinia bibendum nulla sed consectetur. Praesent commodo cursus magna, vel scelerisque nisl consectetur et. Donec sed odio dui. Donec ullamcorper nulla non metus auctor fringilla.</p>
<p>Cras mattis consectetur purus sit amet fermentum. Cras justo odio, dapibus ac facilisis in, egestas eget quam. Morbi leo risus, porta ac consectetur ac, vestibulum at eros.</p>
<p>Praesent commodo cursus magna, vel scelerisque nisl consectetur et. Vivamus sagittis lacus vel augue laoreet rutrum faucibus dolor auctor.</p>
<p>Aenean lacinia bibendum nulla sed consectetur. Praesent commodo cursus magna, vel scelerisque nisl consectetur et. Donec sed odio dui. Donec ullamcorper nulla non metus auctor fringilla.</p>
<p>Cras mattis consectetur purus sit amet fermentum. Cras justo odio, dapibus ac facilisis in, egestas eget quam. Morbi leo risus, porta ac consectetur ac, vestibulum at eros.</p>
<p>Praesent commodo cursus magna, vel scelerisque nisl consectetur et. Vivamus sagittis lacus vel augue laoreet rutrum faucibus dolor auctor.</p>
<p>Aenean lacinia bibendum nulla sed consectetur. Praesent commodo cursus magna, vel scelerisque nisl consectetur et. Donec sed odio dui. Donec ullamcorper nulla non metus auctor fringilla.</p>
</Modal.Body>
<Modal.Footer>
<Button onClick={this.props.onHide}>Close</Button>
</Modal.Footer>
</Modal>
);
}
};
export default LargeModal; |
client/containers/App.js | LinearAtWorst/cogile | import React, { Component } from 'react';
import { connect } from 'react-redux';
import { getUsername, smashUser } from '../actions/index';
import { bindActionCreators } from 'redux';
import NavLink from '../components/NavLink';
import { Link } from 'react-router';
import Radium from 'radium';
import color from 'color';
import helperFunctions from '../utils/helperFunctions';
var RadiumLink = Radium(Link)
class App extends Component {
constructor(props) {
super(props);
}
smash() {
if (helperFunctions.isLoggedIn() === true){
this.props.smashUser();
}
};
render() {
return (
<div className='main-container'>
<nav className="navbar navbar-default">
<div className="container-fluid">
<div className="navbar-collapse navbar-responsive-collapse collapse in" aria-expanded="true">
<RadiumLink
to="/"
onlyActiveOnIndex
className="sitename"
style={styles.base}>nimble<span id="code">code</span>
</RadiumLink>
<ul role="nav" className="nav navbar-nav navbar-right">
<li className="nav-item"><NavLink to="singleplayer/js/00-forLoop" className="nav-label" onlyActiveOnIndex>Singleplayer</NavLink></li>
<li className="nav-item"><NavLink to="multiplayer" className="nav-label">Multiplayer</NavLink></li>
<li className="nav-item"><NavLink to="about" className="nav-label">About</NavLink></li>
{
(helperFunctions.isLoggedIn() === false)
?
<li><NavLink to="login" className="nav-label nav-login">Login</NavLink></li>
:
<li>
<RadiumLink
to="/"
className="nav-label"
onClick={this.smash.bind(this)}>
Logout
</RadiumLink>
</li>
}
</ul>
</div>
</div>
</nav>
{/* Rendering the children of this route below */}
{this.props.children}
</div>
);
}
};
var styles = {
base: {
color: '#eee',
// Adding interactive state couldn't be easier! Add a special key to your
// style object (:hover, :focus, :active, or @media) with the additional rules.
':hover': {
color: color('#eee')
},
':focus': {
color: color('#eee'),
textDecoration: 'none'
}
}
}
function mapStateToProps(state) {
return { SavedUsername: state.SavedUsername }
};
function mapDispatchToProps(dispatch) {
return bindActionCreators({
getUsername: getUsername,
smashUser: smashUser
}, dispatch);
};
export default connect(mapStateToProps, mapDispatchToProps)(App);
|
src/routes/dashboard/components/sales.js | terryli1643/thinkmore | import React from 'react'
import PropTypes from 'prop-types'
import styles from './sales.less'
import classnames from 'classnames'
import { color } from '../../../utils'
import { LineChart, Line, XAxis, YAxis, CartesianGrid, Tooltip, Legend, ResponsiveContainer } from 'recharts'
function Sales ({ data }) {
return (
<div className={styles.sales}>
<div className={styles.title}>Yearly Sales</div>
<ResponsiveContainer minHeight={360}>
<LineChart data={data}>
<Legend verticalAlign="top"
content={prop => {
const { payload } = prop
return (<ul className={classnames({ [styles.legend]: true, clearfix: true })}>
{payload.map((item, key) => <li key={key}><span className={styles.radiusdot} style={{ background: item.color }} />{item.value}</li>)}
</ul>)
}}
/>
<XAxis dataKey="name" axisLine={{ stroke: color.borderBase, strokeWidth: 1 }} tickLine={false} />
<YAxis axisLine={false} tickLine={false} />
<CartesianGrid vertical={false} stroke={color.borderBase} strokeDasharray="3 3" />
<Tooltip
wrapperStyle={{ border: 'none', boxShadow: '4px 4px 40px rgba(0, 0, 0, 0.05)' }}
content={content => {
const list = content.payload.map((item, key) => <li key={key} className={styles.tipitem}><span className={styles.radiusdot} style={{ background: item.color }} />{`${item.name}:${item.value}`}</li>)
return <div className={styles.tooltip}><p className={styles.tiptitle}>{content.label}</p><ul>{list}</ul></div>
}}
/>
<Line type="monotone" dataKey="Food" stroke={color.purple} strokeWidth={3} dot={{ fill: color.purple }} activeDot={{ r: 5, strokeWidth: 0 }} />
<Line type="monotone" dataKey="Clothes" stroke={color.red} strokeWidth={3} dot={{ fill: color.red }} activeDot={{ r: 5, strokeWidth: 0 }} />
<Line type="monotone" dataKey="Electronics" stroke={color.green} strokeWidth={3} dot={{ fill: color.green }} activeDot={{ r: 5, strokeWidth: 0 }} />
</LineChart>
</ResponsiveContainer>
</div>
)
}
Sales.propTypes = {
data: PropTypes.array,
}
export default Sales
|
src/client.js | GetExpert/redux-blog-example | import './css/index.css';
import React from 'react';
import { render } from 'react-dom';
import { AppContainer } from 'react-hot-loader';
import { Provider } from 'react-redux';
import configureStore from './store';
import { BrowserRouter } from 'react-router-dom';
import createRoutes from './routes';
const rootEl = document.getElementById('root');
const App = ({ store }) => {
if (!store) {
let state = null;
try {
state = JSON.parse(window.__INITIAL_STATE__);
} catch (err) {
// TODO send to Sentry
}
store = configureStore(state);
}
const routes = createRoutes(store);
return (
<Provider store={store}>
<BrowserRouter>
{routes}
</BrowserRouter>
</Provider>
);
};
render(
<App />,
rootEl
);
if (module.hot) {
module.hot.accept('./App', () => {
const NextApp = <App />;
render(
<AppContainer>
<NextApp />
</AppContainer>,
rootEl
);
});
}
|
src/components/UI/Dropdown/Dropdown.js | Aloomaio/netlify-cms | import React from 'react';
import PropTypes from 'prop-types';
import c from 'classnames';
import { Wrapper, Button, Menu, MenuItem } from 'react-aria-menubutton';
import { Icon } from 'UI';
const Dropdown = ({
label,
button,
className,
classNameButton = '',
dropdownWidth = 'auto',
dropdownPosition = 'left',
dropdownTopOverlap = '0',
children
}) => {
const style = {
width: dropdownWidth,
top: dropdownTopOverlap,
left: dropdownPosition === 'left' ? 0 : 'auto',
right: dropdownPosition === 'right' ? 0 : 'auto',
};
return (
<Wrapper className={c('nc-dropdown', className)} onSelection={handler => handler()}>
{
button
? <Button>{button}</Button>
: <Button className={c('nc-dropdownButton', classNameButton)}>{label}</Button>
}
<Menu>
<ul className="nc-dropdownList" style={style}>
{children}
</ul>
</Menu>
</Wrapper>
);
};
const DropdownItem = ({ label, icon, iconDirection, onClick, className }) => (
<MenuItem className={c('nc-dropdownItem', className)} value={onClick}>
<span>{label}</span>
{
icon
? <span className="nc-dropdownItemIcon">
<Icon type={icon} direction={iconDirection} size="small"/>
</span>
: null
}
</MenuItem>
);
export { Dropdown, DropdownItem };
|
gadget-system-teamwork/src/components/sub-components/ListCommentPage.js | TeodorDimitrov89/JS-Web-Teamwork-2017 | import React from 'react'
import { Link } from 'react-router-dom'
import Auth from '../users/Auth'
const ListCommentPage = (props) => (
<div className='comments'>
<div className='comment-box'>
<span className='comment-count'>#{props.index}</span>
<span>{props.author} says:</span>
<p>{props.date}</p>
<p>Content: {props.content}</p>
{(Auth.isUserAuthenticated() && Auth.isUserAdmin()) ? (
<div className='list-comments'>
<Link to={`/gadgets/details/delete/comment/${props.commentId}`} className='btn btn-sm btn-danger btn-block' >Delete</Link>
<Link to={`/gadgets/details/edit/comment/${props.commentId}`} className='btn btn-sm btn-info btn-block'>Edit</Link>
</div>
) : '' }
</div>
</div>
)
export default ListCommentPage
|
src/App/Views/Home.js | ccubed/Vertinext | import React from 'react';
class Home extends React.Component {
render() {
return (
<div className="container">
<div className="row">
<div className="col s12">
<div className="card hoverable">
<div className="card-content red-text">
<span className="card-title red-text">Charles C Click</span><br />
Email: <a href="mailto:CharlesClick@vertinext.com">CharlesClick@vertinext.com</a><br />
Skillset: Web Development<br />
This Website: <a href="https://facebook.github.io/react/">React</a> and <a href="http://materializecss.com/">Materialize</a><br />
<blockquote>
I am a coder and web developer in Knoxville, TN, who also hosts websites, games and other services through a personal server. There is a list of my Github repositories and currently hosted games and services available on other pages.
</blockquote>
</div>
<div className="card-action red-text">
<a href="https://docs.google.com/document/d/1ykS2_34-GQd0SbrjpG9NbBvq40L62qWxGJc43KAjOD8/edit?usp=sharing">View Resume</a>
</div>
</div>
</div>
</div>
</div>
);
}
}
module.exports = Home;
|
src/components/run.js | bluebill1049/cart | import React from 'react';
import ReactDOM from 'react-dom';
import App from './Main';
// Render the main component into the dom
ReactDOM.render(<App />, document.getElementById('app'));
|
src/app/contentItem.js | approximata/theUltimateWeddingPage | import React from 'react'
import PropTypes from 'prop-types'
import TableItem from './tableItems'
const ContentItem = ({ item }) => {
let rows = item.rows
let table = []
table = rows.map((itemElem, index) =>
(<TableItem item={itemElem} key={index}/>))
return (
<section className={item.title}>
<table className='table table-border'><tbody>{table}</tbody></table>
<div name={ item.subTitle1 } className={item.title + ' subTitle1' + ' container'}>
<h2><a href={item.link}><span className={ item.icon }></span></a>{item.subTitle1}</h2>
<p>{item.content1}</p>
</div>
<div name={ item.subTitle2 } className={item.title + ' subTitle2' + ' container'}>
<h2>{item.subTitle2}</h2>
<p>{item.content2}</p>
</div>
</section>
)
}
ContentItem.propTypes = {
item: PropTypes.object
}
export default ContentItem
|
examples/js/advance/insert-type-table.js | prajapati-parth/react-bootstrap-table | /* eslint max-len: 0 */
import React from 'react';
import { BootstrapTable, TableHeaderColumn } from 'react-bootstrap-table';
const jobs = [];
const jobTypes = [ 'A', 'B', 'C', 'D' ];
function addJobs(quantity) {
const startId = jobs.length;
for (let i = 0; i < quantity; i++) {
const id = startId + i;
jobs.push({
id: id,
name: 'Item name ' + id,
type: 'B',
active: i % 2 === 0 ? 'Y' : 'N'
});
}
}
addJobs(5);
export default class DataInsertTypeTable extends React.Component {
render() {
return (
<BootstrapTable data={ jobs } insertRow={ true }>
<TableHeaderColumn dataField='id' isKey={ true }>Job ID</TableHeaderColumn>
<TableHeaderColumn dataField='name' editable={ { type: 'textarea' } }>Job Name</TableHeaderColumn>
<TableHeaderColumn dataField='type' editable={ { type: 'select', options: { values: jobTypes } } }>Job Type</TableHeaderColumn>
<TableHeaderColumn dataField='active' editable={ { type: 'checkbox', options: { values: 'Y:N' } } }>Active</TableHeaderColumn>
</BootstrapTable>
);
}
}
|
src/components/atoms/Dialog/DialogActions.js | ygoto3/artwork-manager | // @flow
import React, { Component } from 'react';
import { Button } from '../../atoms/Button';
export type DialogAction = {
label: string;
onClick: Function;
};
export type DialogActionsProps = {
actions: DialogAction[];
onClose: Function;
}
export class DialogActions extends Component<*, DialogActionsProps, *> {
render() {
const { actions, onClose } = this.props;
return (
<div>
{actions.map((a, i) => (
<Button key={i} onClick={a.onClick}>{a.label}</Button>
))}
<Button onClick={onClose}>Close</Button>
</div>
);
}
}
Object.assign(DialogActions, {
defaultProps: {
actions: []
},
});
|
src/index.js | plus-n-boots/plus-n-boots.github.io | import React from 'react'
import AppWrapper from './containers/AppWrapper'
import 'material-design-lite/material.css'
React.render(
<AppWrapper />,
document.querySelector('wrapper')
)
|
src/components/Table.js | cardotrejos/calculator | import React from 'react';
export default ({headings, rows, totals, className, style})=> (
<table className={className} style={style}>
<thead>
<tr>
{headings.map((d,i)=><th key={i}>{d}</th>)}
</tr>
</thead>
<tbody>
{rows.map((row,index)=>(
<tr key={index}>
{row.map((d,i)=><td key={i}>{d.toLocaleString()}</td>)}
</tr>)
)
}
</tbody>
<tfoot>
<tr>
{totals.map((d,i)=><td key={i}>{d.toLocaleString()}</td>)}
</tr>
</tfoot>
</table>
);
|
No dataset card yet