content
stringlengths 5
1.03M
| input_ids
sequencelengths 4
823k
| ratio_char_token
float64 0.4
12.5
| token_count
int64 4
823k
|
---|---|---|---|
using HetaSimulator, Plots
p = load_platform(".", rm_out=false)
scenarios = read_scenarios("data-mumenthaler-2000/scenarios.csv")
add_scenarios!(p, scenarios)
data = read_measurements("data-mumenthaler-2000/data.csv")
data_scn = Dict()
data_scn[:scn1] = filter(:scenario => ==(:scn1), data)
data_scn[:scn2] = filter(:scenario => ==(:scn2), data)
data_scn[:scn3] = filter(:scenario => ==(:scn3), data)
loss_add = Dict()
loss_add[:scn1] = 2*sum(log.(data_scn[:scn1][:,"prob.sigma"])) + length(data_scn[:scn1][:,"prob.sigma"])*log(2π)
loss_add[:scn2] = 2*sum(log.(data_scn[:scn2][:,"prob.sigma"])) + length(data_scn[:scn1][:,"prob.sigma"])*log(2π)
loss_add[:scn3] = 2*sum(log.(data_scn[:scn3][:,"prob.sigma"])) + length(data_scn[:scn1][:,"prob.sigma"])*log(2π)
add_measurements!(p, data)
# initial plot
sim(p) |> plot
sim(p, parameters_upd = [:Vmax=>0.3, :ke=>0., :k_a=>5.]) |> plot
# fitting 1 best -63.45
params_df_1 = read_parameters("./julia/parameters-1.csv")
res_fit_1 = fit(p, params_df_1; scenarios=[:scn1], ftol_abs=1e-6, ftol_rel=0.)
res_fit_1 = fit(p, optim(res_fit_1); scenarios=[:scn1], ftol_abs=1e-6, ftol_rel=0.)
fig = sim(p, scenarios=[:scn1], parameters_upd=optim(res_fit_1)) |> plot
# savefig(fig, "diagnostics/scn1_best.png")
# fitting 2 best -63.64
params_df_2 = read_parameters("./julia/parameters-2.csv")
res_fit_2 = fit(p, params_df_2; scenarios=[:scn2], ftol_abs=1e-6, ftol_rel=0.)
res_fit_2 = fit(p, optim(res_fit_2); scenarios=[:scn2], ftol_abs=1e-6, ftol_rel=0.)
fig = sim(p, scenarios=[:scn2], parameters_upd=optim(res_fit_2)) |> plot
# savefig(fig, "diagnostics/scn2_best.png")
# fitting 3 best -63.92
params_df_3 = read_parameters("./julia/parameters-3.csv")
res_fit_3 = fit(p, params_df_3; scenarios=[:scn3], ftol_abs=1e-6, ftol_rel=0.)
res_fit_3 = fit(p, optim(res_fit_3); scenarios=[:scn3], ftol_abs=1e-6, ftol_rel=0.)
fig = sim(p, scenarios=[:scn3], parameters_upd=optim(res_fit_3)) |> plot
# savefig(fig, "diagnostics/scn3_best.png")
############################## Identification ##########################
using LikelihoodProfiler, CSV
chi_level = 3.84
p_optim_1 = optim(res_fit_1)
p_optim_2 = optim(res_fit_2)
p_optim_3 = optim(res_fit_3)
sim_scn1 = sim(p.scenarios[:scn1], parameters_upd=p_optim_1)
sim_scn2 = sim(p.scenarios[:scn2], parameters_upd=p_optim_2)
sim_scn3 = sim(p.scenarios[:scn3], parameters_upd=p_optim_3)
p_names = Dict(
:scn1 => first.(p_optim_1),
:scn2 => first.(p_optim_2),
:scn3 => first.(p_optim_3),
)
function loss_func(params::Vector{P}, scen) where P<: Pair
sim_res = sim(p.scenarios[scen]; parameters_upd=params, reltol=1e-6, abstol=1e-8)
#sim_res = last(sim_vec[1])
return loss(sim_res, sim_res.scenario.measurements) - loss_add[scen]
end
function loss_func(pvals::Vector{N}, scen) where N <: Number
# @show pvals
@assert length(p_names[scen]) == length(pvals) "Number of params values doesn't match params names"
params = [pn => pv for (pn,pv) in zip(p_names[scen],pvals)]
loss_func(params,scen)
end
loss_scn1(params) = loss_func(params, :scn1)
loss_scn2(params) = loss_func(params, :scn2)
loss_scn3(params) = loss_func(params, :scn3)
function scan_func(params::Vector{P}, timepoint, scen) where P<: Pair
sim_vec = sim(p; parameters_upd=params, scenarios=[scen])
return last(sim_vec[1])(timepoint)[:BrAC]
end
function scan_func(pvals::Vector{N}, timepoint, scen) where N <: Number
@assert length(p_names[scen]) == length(pvals) "Number of params values doesn't match params names"
params = [pn => pv for (pn,pv) in zip(p_names[scen],pvals)]
scan_func(params, timepoint, scen)
end
scan_scn1(params, timepoint) = scan_func(params, timepoint, :scn1)
scan_scn2(params, timepoint) = scan_func(params, timepoint, :scn2)
scan_scn3(params, timepoint) = scan_func(params, timepoint, :scn3)
saveat_1 = saveat(p.scenarios[:scn1])
saveat_2 = saveat(p.scenarios[:scn2])
saveat_3 = saveat(p.scenarios[:scn3])
p_ident_1 = [get_interval(
last.(p_optim_1),
i,
loss_scn1,
:CICO_ONE_PASS,
theta_bounds = fill((1e-10,1e10), length(p_names[:scn1])),
scan_bounds=((last.(p_optim_1)[i])/1e4, (last.(p_optim_1)[i])*1e4),
scan_tol=1e-5,
#scale = fill(:log, length(p_names[:scn1])),
loss_crit = loss_scn1(p_optim_1) + chi_level
) for i in eachindex(p_names[:scn1])]
plb_1 = [iv.result[1].value for iv in p_ident_1]
pub_1 = [iv.result[2].value for iv in p_ident_1]
pliter_1 = [iv.result[1].counter for iv in p_ident_1]
puiter_1 = [iv.result[2].counter for iv in p_ident_1]
df = DataFrame(params = p_names[:scn1], optim = last.(p_optim_1), lower = plb_1, upper = pub_1, liter = pliter_1, uiter = puiter_1)
df.lower = replace(df.lower, nothing => missing)
df.upper = replace(df.upper, nothing => missing)
CSV.write("./julia/scn1_intervals.csv", df)
p_ident_2 = [get_interval(
last.(p_optim_2),
i,
loss_scn2,
:CICO_ONE_PASS,
#theta_bounds = fill((-10.,10.), length(p_names[:scn2])),
#scale = fill(:log, length(p_names[:scn2])),
loss_crit = loss_scn2(p_optim_2) + chi_level
) for i in eachindex(p_names[:scn2])]
p_ident_3 = [get_interval(
last.(p_optim_3),
i,
loss_scn3,
:CICO_ONE_PASS,
scale = fill(:log, length(p_names[:scn3])),
loss_crit = loss_scn3(p_optim_3) + chi_level
) for i in eachindex(p_names[:scn3])]
BrAC_ident_1 = [get_interval(
last.(p_optim_1),
params->scan_scn1(params,t),
loss_scn1,
:CICO_ONE_PASS,
scale = fill(:log, length(p_names[:scn1])),
loss_crit = loss_scn1(p_optim_1) + chi_level
) for t in saveat_1]
BrAC_ident_2 = [get_interval(
last.(p_optim_2),
params->scan_scn2(params,t),
loss_scn2,
:CICO_ONE_PASS,
scale = fill(:log, length(p_names[:scn2])),
loss_crit = loss_scn2(p_optim_2) + chi_level
) for t in saveat_2]
BrAC_ident_3 = [get_interval(
last.(p_optim_3),
params->scan_scn3(params,t),
loss_scn3,
:CICO_ONE_PASS,
scale = fill(:log, length(p_names[:scn3])),
loss_crit = loss_scn3(p_optim_3) + chi_level
) for t in saveat_3]
lb_1 = [iv.result[1].value for iv in BrAC_ident_1]
ub_1 = [iv.result[2].value for iv in BrAC_ident_1]
liter_1 = [iv.result[1].counter for iv in BrAC_ident_1]
uiter_1 = [iv.result[2].counter for iv in BrAC_ident_1]
df = DataFrame(times = saveat_1, lower = lb_1, upper = ub_1, liter = liter_1, uiter = uiter_1)
df.lower = replace(df.lower, nothing => missing)
df.upper = replace(df.upper, nothing => missing)
CSV.write("./julia/scn1_conf_band.csv", df)
BrAC_1 = sim_scn1.(saveat_1, :BrAC)
plot(sim_scn1, show_measurements=false, vars=[:BrAC])
scatter!(data_scn[:scn1].t, data_scn[:scn1].measurement, yerror=data_scn[:scn1][!,"prob.sigma"], label = "Measurements")
plot!(saveat_1, lb_1, fillrange = ub_1, fillalpha = 0.35, c = 1, label = "Confidence band")
savefig("./julia/conf_band.png")
lb_2 = [iv.result[1].value for iv in BrAC_ident_2]
ub_2 = [iv.result[2].value for iv in BrAC_ident_2]
BrAC_2 = sim_scn2.(saveat_2, :BrAC)
plot(sim_scn2, show_measurements=false, ribbon = (BrAC_2-lb_1,ub_2-BrAC_2), fc=:orange, fa=0.7)
lb_3 = [iv.result[1].value for iv in BrAC_ident_3]
ub_3 = [iv.result[2].value for iv in BrAC_ident_3]
BrAC_3 = sim_scn3.(saveat_3, :BrAC)
plot(sim_scn3, show_measurements=false, ribbon = (BrAC_3-lb_3,ub_3-BrAC_3), fc=:orange, fa=0.7)
### Validation band
t_scn1 = data_scn[:scn1].t
sigma_scn1 = data_scn[:scn1][!,"prob.sigma"]
sim_scn1 = sim(p.scenarios[:scn1]; parameters_upd=p_optim_1, reltol=1e-6, abstol=1e-8)
BrAC_scn1 = sim_scn1.(t_scn1, :BrAC)
function valid_obj1(params, i)
d1 = last(params)
_params = [pn => pv for (pn,pv) in zip(p_names[:scn1],params[1:end-1])]
sim_res = sim(p.scenarios[:scn1]; parameters_upd=_params, reltol=1e-6, abstol=1e-8)
d1_sim = sim_res(t_scn1[i])[:BrAC]
return loss(sim_res, sim_res.scenario.measurements) + (d1 - d1_sim)^2/(sigma_scn1[i])^2 - loss_add[:scn1]
end
valid_1 = []
for i in eachindex(t_scn1)
println(" Calculating CI for $(t_scn1[i]) timepoint")
push!(valid_1,
get_interval(
[last.(p_optim_1); BrAC_scn1[i]],
5,
p->valid_obj1(p,i),
:CICO_ONE_PASS,
theta_bounds = fill((1e-8,1e8), length(p_names[:scn1])+1),
scan_bounds=(1e-7,1e7),
scan_tol=1e-5,
scale = fill(:log, length(p_names[:scn1])+1),
loss_crit = loss_scn1(p_optim_1) + chi_level)
)
end
lb_1 = [iv.result[1].value for iv in valid_1]
ub_1 = [iv.result[2].value for iv in valid_1]
liter_1 = [iv.result[1].counter for iv in valid_1]
uiter_1 = [iv.result[2].counter for iv in valid_1]
df = DataFrame(times = t_scn1, lower = lb_1, upper = ub_1, liter = liter_1, uiter = uiter_1)
df.lower = replace(df.lower, nothing => missing)
df.upper = replace(df.upper, nothing => missing)
CSV.write("./julia/scn1_valid_bans.csv", df)
lb_1[1] = 0.0
lb_1[end-4:end] .= 0.0
plot(sim_scn1, show_measurements=false, vars=[:BrAC])
scatter!(data_scn[:scn1].t, data_scn[:scn1].measurement, yerror=data_scn[:scn1][!,"prob.sigma"], label = "Measurements")
plot!(t_scn1, lb_1, fillrange = ub_1, fillalpha = 0.35, c = 1, label = "Validation band")
savefig("./julia/valid_band.png") | [
3500,
367,
17167,
8890,
8927,
11,
1345,
1747,
198,
198,
79,
796,
3440,
62,
24254,
7203,
33283,
42721,
62,
448,
28,
9562,
8,
198,
198,
1416,
268,
13010,
796,
1100,
62,
1416,
268,
13010,
7203,
7890,
12,
76,
42300,
263,
12,
11024,
14,
1416,
268,
13010,
13,
40664,
4943,
198,
2860,
62,
1416,
268,
13010,
0,
7,
79,
11,
13858,
8,
198,
198,
7890,
796,
1100,
62,
1326,
5015,
902,
7203,
7890,
12,
76,
42300,
263,
12,
11024,
14,
7890,
13,
40664,
4943,
198,
7890,
62,
1416,
77,
796,
360,
713,
3419,
198,
7890,
62,
1416,
77,
58,
25,
1416,
77,
16,
60,
796,
8106,
7,
25,
1416,
39055,
5218,
6624,
7,
25,
1416,
77,
16,
828,
1366,
8,
198,
7890,
62,
1416,
77,
58,
25,
1416,
77,
17,
60,
796,
8106,
7,
25,
1416,
39055,
5218,
6624,
7,
25,
1416,
77,
17,
828,
1366,
8,
198,
7890,
62,
1416,
77,
58,
25,
1416,
77,
18,
60,
796,
8106,
7,
25,
1416,
39055,
5218,
6624,
7,
25,
1416,
77,
18,
828,
1366,
8,
198,
198,
22462,
62,
2860,
796,
360,
713,
3419,
198,
22462,
62,
2860,
58,
25,
1416,
77,
16,
60,
796,
362,
9,
16345,
7,
6404,
12195,
7890,
62,
1416,
77,
58,
25,
1416,
77,
16,
7131,
25,
553,
1676,
65,
13,
82,
13495,
8973,
4008,
1343,
4129,
7,
7890,
62,
1416,
77,
58,
25,
1416,
77,
16,
7131,
25,
553,
1676,
65,
13,
82,
13495,
8973,
27493,
6404,
7,
17,
46582,
8,
198,
22462,
62,
2860,
58,
25,
1416,
77,
17,
60,
796,
362,
9,
16345,
7,
6404,
12195,
7890,
62,
1416,
77,
58,
25,
1416,
77,
17,
7131,
25,
553,
1676,
65,
13,
82,
13495,
8973,
4008,
1343,
4129,
7,
7890,
62,
1416,
77,
58,
25,
1416,
77,
16,
7131,
25,
553,
1676,
65,
13,
82,
13495,
8973,
27493,
6404,
7,
17,
46582,
8,
198,
22462,
62,
2860,
58,
25,
1416,
77,
18,
60,
796,
362,
9,
16345,
7,
6404,
12195,
7890,
62,
1416,
77,
58,
25,
1416,
77,
18,
7131,
25,
553,
1676,
65,
13,
82,
13495,
8973,
4008,
1343,
4129,
7,
7890,
62,
1416,
77,
58,
25,
1416,
77,
16,
7131,
25,
553,
1676,
65,
13,
82,
13495,
8973,
27493,
6404,
7,
17,
46582,
8,
198,
198,
2860,
62,
1326,
5015,
902,
0,
7,
79,
11,
1366,
8,
198,
198,
2,
4238,
7110,
198,
14323,
7,
79,
8,
930,
29,
7110,
198,
14323,
7,
79,
11,
10007,
62,
929,
67,
796,
685,
25,
53,
9806,
14804,
15,
13,
18,
11,
1058,
365,
14804,
15,
1539,
1058,
74,
62,
64,
14804,
20,
8183,
8,
930,
29,
7110,
198,
198,
2,
15830,
352,
1266,
532,
5066,
13,
2231,
198,
37266,
62,
7568,
62,
16,
796,
1100,
62,
17143,
7307,
7,
1911,
14,
73,
43640,
14,
17143,
7307,
12,
16,
13,
40664,
4943,
198,
411,
62,
11147,
62,
16,
796,
4197,
7,
79,
11,
42287,
62,
7568,
62,
16,
26,
13858,
41888,
25,
1416,
77,
16,
4357,
10117,
349,
62,
8937,
28,
16,
68,
12,
21,
11,
10117,
349,
62,
2411,
28,
15,
2014,
198,
411,
62,
11147,
62,
16,
796,
4197,
7,
79,
11,
6436,
7,
411,
62,
11147,
62,
16,
1776,
13858,
41888,
25,
1416,
77,
16,
4357,
10117,
349,
62,
8937,
28,
16,
68,
12,
21,
11,
10117,
349,
62,
2411,
28,
15,
2014,
198,
5647,
796,
985,
7,
79,
11,
13858,
41888,
25,
1416,
77,
16,
4357,
10007,
62,
929,
67,
28,
40085,
7,
411,
62,
11147,
62,
16,
4008,
930,
29,
7110,
198,
2,
3613,
5647,
7,
5647,
11,
366,
47356,
34558,
14,
1416,
77,
16,
62,
13466,
13,
11134,
4943,
628,
198,
2,
15830,
362,
1266,
532,
5066,
13,
2414,
198,
37266,
62,
7568,
62,
17,
796,
1100,
62,
17143,
7307,
7,
1911,
14,
73,
43640,
14,
17143,
7307,
12,
17,
13,
40664,
4943,
198,
411,
62,
11147,
62,
17,
796,
4197,
7,
79,
11,
42287,
62,
7568,
62,
17,
26,
13858,
41888,
25,
1416,
77,
17,
4357,
10117,
349,
62,
8937,
28,
16,
68,
12,
21,
11,
10117,
349,
62,
2411,
28,
15,
2014,
198,
411,
62,
11147,
62,
17,
796,
4197,
7,
79,
11,
6436,
7,
411,
62,
11147,
62,
17,
1776,
13858,
41888,
25,
1416,
77,
17,
4357,
10117,
349,
62,
8937,
28,
16,
68,
12,
21,
11,
10117,
349,
62,
2411,
28,
15,
2014,
198,
5647,
796,
985,
7,
79,
11,
13858,
41888,
25,
1416,
77,
17,
4357,
10007,
62,
929,
67,
28,
40085,
7,
411,
62,
11147,
62,
17,
4008,
930,
29,
7110,
198,
2,
3613,
5647,
7,
5647,
11,
366,
47356,
34558,
14,
1416,
77,
17,
62,
13466,
13,
11134,
4943,
628,
198,
2,
15830,
513,
1266,
532,
5066,
13,
5892,
198,
37266,
62,
7568,
62,
18,
796,
1100,
62,
17143,
7307,
7,
1911,
14,
73,
43640,
14,
17143,
7307,
12,
18,
13,
40664,
4943,
198,
411,
62,
11147,
62,
18,
796,
4197,
7,
79,
11,
42287,
62,
7568,
62,
18,
26,
13858,
41888,
25,
1416,
77,
18,
4357,
10117,
349,
62,
8937,
28,
16,
68,
12,
21,
11,
10117,
349,
62,
2411,
28,
15,
2014,
198,
411,
62,
11147,
62,
18,
796,
4197,
7,
79,
11,
6436,
7,
411,
62,
11147,
62,
18,
1776,
13858,
41888,
25,
1416,
77,
18,
4357,
10117,
349,
62,
8937,
28,
16,
68,
12,
21,
11,
10117,
349,
62,
2411,
28,
15,
2014,
198,
5647,
796,
985,
7,
79,
11,
13858,
41888,
25,
1416,
77,
18,
4357,
10007,
62,
929,
67,
28,
40085,
7,
411,
62,
11147,
62,
18,
4008,
930,
29,
7110,
198,
2,
3613,
5647,
7,
5647,
11,
366,
47356,
34558,
14,
1416,
77,
18,
62,
13466,
13,
11134,
4943,
198,
198,
14468,
7804,
4242,
2235,
38657,
1303,
14468,
7804,
2,
198,
198,
3500,
4525,
11935,
15404,
5329,
11,
44189,
198,
198,
11072,
62,
5715,
796,
513,
13,
5705,
198,
198,
79,
62,
40085,
62,
16,
796,
6436,
7,
411,
62,
11147,
62,
16,
8,
198,
79,
62,
40085,
62,
17,
796,
6436,
7,
411,
62,
11147,
62,
17,
8,
198,
79,
62,
40085,
62,
18,
796,
6436,
7,
411,
62,
11147,
62,
18,
8,
198,
198,
14323,
62,
1416,
77,
16,
796,
985,
7,
79,
13,
1416,
268,
13010,
58,
25,
1416,
77,
16,
4357,
10007,
62,
929,
67,
28,
79,
62,
40085,
62,
16,
8,
198,
14323,
62,
1416,
77,
17,
796,
985,
7,
79,
13,
1416,
268,
13010,
58,
25,
1416,
77,
17,
4357,
10007,
62,
929,
67,
28,
79,
62,
40085,
62,
17,
8,
198,
14323,
62,
1416,
77,
18,
796,
985,
7,
79,
13,
1416,
268,
13010,
58,
25,
1416,
77,
18,
4357,
10007,
62,
929,
67,
28,
79,
62,
40085,
62,
18,
8,
198,
198,
79,
62,
14933,
796,
360,
713,
7,
198,
220,
1058,
1416,
77,
16,
5218,
717,
12195,
79,
62,
40085,
62,
16,
828,
198,
220,
1058,
1416,
77,
17,
5218,
717,
12195,
79,
62,
40085,
62,
17,
828,
198,
220,
1058,
1416,
77,
18,
5218,
717,
12195,
79,
62,
40085,
62,
18,
828,
198,
8,
198,
198,
8818,
2994,
62,
20786,
7,
37266,
3712,
38469,
90,
47,
5512,
4408,
8,
810,
350,
27,
25,
39645,
198,
220,
985,
62,
411,
796,
985,
7,
79,
13,
1416,
268,
13010,
58,
1416,
268,
11208,
10007,
62,
929,
67,
28,
37266,
11,
823,
83,
349,
28,
16,
68,
12,
21,
11,
16552,
349,
28,
16,
68,
12,
23,
8,
198,
220,
1303,
14323,
62,
411,
796,
938,
7,
14323,
62,
35138,
58,
16,
12962,
198,
220,
1441,
2994,
7,
14323,
62,
411,
11,
985,
62,
411,
13,
1416,
39055,
13,
1326,
5015,
902,
8,
532,
2994,
62,
2860,
58,
1416,
268,
60,
198,
437,
198,
198,
8818,
2994,
62,
20786,
7,
79,
12786,
3712,
38469,
90,
45,
5512,
4408,
8,
810,
399,
1279,
25,
7913,
198,
1303,
2488,
12860,
279,
12786,
198,
220,
2488,
30493,
4129,
7,
79,
62,
14933,
58,
1416,
268,
12962,
6624,
4129,
7,
79,
12786,
8,
366,
15057,
286,
42287,
3815,
1595,
470,
2872,
42287,
3891,
1,
198,
220,
42287,
796,
685,
21999,
5218,
279,
85,
329,
357,
21999,
11,
79,
85,
8,
287,
19974,
7,
79,
62,
14933,
58,
1416,
268,
4357,
79,
12786,
15437,
198,
220,
2994,
62,
20786,
7,
37266,
11,
1416,
268,
8,
198,
437,
198,
198,
22462,
62,
1416,
77,
16,
7,
37266,
8,
796,
2994,
62,
20786,
7,
37266,
11,
1058,
1416,
77,
16,
8,
198,
22462,
62,
1416,
77,
17,
7,
37266,
8,
796,
2994,
62,
20786,
7,
37266,
11,
1058,
1416,
77,
17,
8,
198,
22462,
62,
1416,
77,
18,
7,
37266,
8,
796,
2994,
62,
20786,
7,
37266,
11,
1058,
1416,
77,
18,
8,
198,
198,
8818,
9367,
62,
20786,
7,
37266,
3712,
38469,
90,
47,
5512,
640,
4122,
11,
4408,
8,
810,
350,
27,
25,
39645,
198,
220,
985,
62,
35138,
796,
985,
7,
79,
26,
10007,
62,
929,
67,
28,
37266,
11,
13858,
41888,
1416,
268,
12962,
198,
220,
1441,
938,
7,
14323,
62,
35138,
58,
16,
60,
5769,
2435,
4122,
38381,
25,
9414,
2246,
60,
198,
437,
198,
198,
8818,
9367,
62,
20786,
7,
79,
12786,
3712,
38469,
90,
45,
5512,
640,
4122,
11,
4408,
8,
810,
399,
1279,
25,
7913,
198,
220,
2488,
30493,
4129,
7,
79,
62,
14933,
58,
1416,
268,
12962,
6624,
4129,
7,
79,
12786,
8,
366,
15057,
286,
42287,
3815,
1595,
470,
2872,
42287,
3891,
1,
198,
220,
42287,
796,
685,
21999,
5218,
279,
85,
329,
357,
21999,
11,
79,
85,
8,
287,
19974,
7,
79,
62,
14933,
58,
1416,
268,
4357,
79,
12786,
15437,
198,
220,
9367,
62,
20786,
7,
37266,
11,
640,
4122,
11,
4408,
8,
198,
437,
198,
198,
35836,
62,
1416,
77,
16,
7,
37266,
11,
640,
4122,
8,
796,
9367,
62,
20786,
7,
37266,
11,
640,
4122,
11,
1058,
1416,
77,
16,
8,
198,
35836,
62,
1416,
77,
17,
7,
37266,
11,
640,
4122,
8,
796,
9367,
62,
20786,
7,
37266,
11,
640,
4122,
11,
1058,
1416,
77,
17,
8,
198,
35836,
62,
1416,
77,
18,
7,
37266,
11,
640,
4122,
8,
796,
9367,
62,
20786,
7,
37266,
11,
640,
4122,
11,
1058,
1416,
77,
18,
8,
198,
198,
21928,
265,
62,
16,
796,
3613,
265,
7,
79,
13,
1416,
268,
13010,
58,
25,
1416,
77,
16,
12962,
198,
21928,
265,
62,
17,
796,
3613,
265,
7,
79,
13,
1416,
268,
13010,
58,
25,
1416,
77,
17,
12962,
198,
21928,
265,
62,
18,
796,
3613,
265,
7,
79,
13,
1416,
268,
13010,
58,
25,
1416,
77,
18,
12962,
198,
198,
79,
62,
738,
62,
16,
796,
685,
1136,
62,
3849,
2100,
7,
198,
220,
938,
12195,
79,
62,
40085,
62,
16,
828,
198,
220,
1312,
11,
198,
220,
2994,
62,
1416,
77,
16,
11,
198,
220,
1058,
34,
22707,
62,
11651,
62,
47924,
11,
198,
220,
262,
8326,
62,
65,
3733,
796,
6070,
19510,
16,
68,
12,
940,
11,
16,
68,
940,
828,
4129,
7,
79,
62,
14933,
58,
25,
1416,
77,
16,
12962,
828,
198,
220,
9367,
62,
65,
3733,
16193,
7,
12957,
12195,
79,
62,
40085,
62,
16,
38381,
72,
12962,
14,
16,
68,
19,
11,
357,
12957,
12195,
79,
62,
40085,
62,
16,
38381,
72,
12962,
9,
16,
68,
19,
828,
198,
220,
9367,
62,
83,
349,
28,
16,
68,
12,
20,
11,
198,
220,
1303,
9888,
796,
220,
6070,
7,
25,
6404,
11,
4129,
7,
79,
62,
14933,
58,
25,
1416,
77,
16,
12962,
828,
198,
220,
2994,
62,
22213,
796,
2994,
62,
1416,
77,
16,
7,
79,
62,
40085,
62,
16,
8,
1343,
33166,
62,
5715,
198,
220,
1267,
329,
1312,
287,
1123,
9630,
7,
79,
62,
14933,
58,
25,
1416,
77,
16,
12962,
60,
198,
198,
489,
65,
62,
16,
796,
685,
452,
13,
20274,
58,
16,
4083,
8367,
329,
21628,
287,
279,
62,
738,
62,
16,
60,
198,
12984,
62,
16,
796,
685,
452,
13,
20274,
58,
17,
4083,
8367,
329,
21628,
287,
279,
62,
738,
62,
16,
60,
198,
198,
489,
2676,
62,
16,
796,
685,
452,
13,
20274,
58,
16,
4083,
24588,
329,
21628,
287,
279,
62,
738,
62,
16,
60,
198,
19944,
2676,
62,
16,
796,
685,
452,
13,
20274,
58,
17,
4083,
24588,
329,
21628,
287,
279,
62,
738,
62,
16,
60,
198,
198,
7568,
796,
6060,
19778,
7,
37266,
796,
279,
62,
14933,
58,
25,
1416,
77,
16,
4357,
6436,
796,
938,
12195,
79,
62,
40085,
62,
16,
828,
2793,
796,
458,
65,
62,
16,
11,
6727,
796,
2240,
62,
16,
11,
4187,
796,
458,
2676,
62,
16,
11,
334,
2676,
796,
47574,
2676,
62,
16,
8,
198,
7568,
13,
21037,
796,
6330,
7,
7568,
13,
21037,
11,
2147,
5218,
4814,
8,
198,
7568,
13,
45828,
796,
6330,
7,
7568,
13,
45828,
11,
2147,
5218,
4814,
8,
198,
7902,
53,
13,
13564,
7,
1911,
14,
73,
43640,
14,
1416,
77,
16,
62,
3849,
12786,
13,
40664,
1600,
47764,
8,
198,
198,
79,
62,
738,
62,
17,
796,
685,
1136,
62,
3849,
2100,
7,
198,
220,
938,
12195,
79,
62,
40085,
62,
17,
828,
198,
220,
1312,
11,
198,
220,
2994,
62,
1416,
77,
17,
11,
198,
220,
1058,
34,
22707,
62,
11651,
62,
47924,
11,
198,
220,
1303,
1169,
8326,
62,
65,
3733,
796,
6070,
19510,
12,
940,
1539,
940,
12179,
4129,
7,
79,
62,
14933,
58,
25,
1416,
77,
17,
12962,
828,
198,
220,
1303,
9888,
796,
6070,
7,
25,
6404,
11,
4129,
7,
79,
62,
14933,
58,
25,
1416,
77,
17,
12962,
828,
198,
220,
2994,
62,
22213,
796,
2994,
62,
1416,
77,
17,
7,
79,
62,
40085,
62,
17,
8,
1343,
33166,
62,
5715,
198,
8,
329,
1312,
287,
1123,
9630,
7,
79,
62,
14933,
58,
25,
1416,
77,
17,
12962,
60,
198,
198,
79,
62,
738,
62,
18,
796,
685,
1136,
62,
3849,
2100,
7,
198,
220,
938,
12195,
79,
62,
40085,
62,
18,
828,
198,
220,
1312,
11,
198,
220,
2994,
62,
1416,
77,
18,
11,
198,
220,
1058,
34,
22707,
62,
11651,
62,
47924,
11,
198,
220,
5046,
796,
6070,
7,
25,
6404,
11,
4129,
7,
79,
62,
14933,
58,
25,
1416,
77,
18,
12962,
828,
198,
220,
2994,
62,
22213,
796,
2994,
62,
1416,
77,
18,
7,
79,
62,
40085,
62,
18,
8,
1343,
33166,
62,
5715,
198,
8,
329,
1312,
287,
1123,
9630,
7,
79,
62,
14933,
58,
25,
1416,
77,
18,
12962,
60,
198,
198,
9414,
2246,
62,
738,
62,
16,
796,
685,
1136,
62,
3849,
2100,
7,
198,
220,
938,
12195,
79,
62,
40085,
62,
16,
828,
198,
220,
42287,
3784,
35836,
62,
1416,
77,
16,
7,
37266,
11,
83,
828,
198,
220,
2994,
62,
1416,
77,
16,
11,
198,
220,
1058,
34,
22707,
62,
11651,
62,
47924,
11,
198,
220,
5046,
796,
6070,
7,
25,
6404,
11,
4129,
7,
79,
62,
14933,
58,
25,
1416,
77,
16,
12962,
828,
198,
220,
2994,
62,
22213,
796,
2994,
62,
1416,
77,
16,
7,
79,
62,
40085,
62,
16,
8,
1343,
33166,
62,
5715,
198,
8,
329,
256,
287,
3613,
265,
62,
16,
60,
198,
198,
9414,
2246,
62,
738,
62,
17,
796,
685,
1136,
62,
3849,
2100,
7,
198,
220,
938,
12195,
79,
62,
40085,
62,
17,
828,
198,
220,
42287,
3784,
35836,
62,
1416,
77,
17,
7,
37266,
11,
83,
828,
198,
220,
2994,
62,
1416,
77,
17,
11,
198,
220,
1058,
34,
22707,
62,
11651,
62,
47924,
11,
198,
220,
5046,
796,
6070,
7,
25,
6404,
11,
4129,
7,
79,
62,
14933,
58,
25,
1416,
77,
17,
12962,
828,
198,
220,
2994,
62,
22213,
796,
2994,
62,
1416,
77,
17,
7,
79,
62,
40085,
62,
17,
8,
1343,
33166,
62,
5715,
198,
8,
329,
256,
287,
3613,
265,
62,
17,
60,
198,
198,
9414,
2246,
62,
738,
62,
18,
796,
685,
1136,
62,
3849,
2100,
7,
198,
220,
938,
12195,
79,
62,
40085,
62,
18,
828,
198,
220,
42287,
3784,
35836,
62,
1416,
77,
18,
7,
37266,
11,
83,
828,
198,
220,
2994,
62,
1416,
77,
18,
11,
198,
220,
1058,
34,
22707,
62,
11651,
62,
47924,
11,
198,
220,
5046,
796,
6070,
7,
25,
6404,
11,
4129,
7,
79,
62,
14933,
58,
25,
1416,
77,
18,
12962,
828,
198,
220,
2994,
62,
22213,
796,
2994,
62,
1416,
77,
18,
7,
79,
62,
40085,
62,
18,
8,
1343,
33166,
62,
5715,
198,
8,
329,
256,
287,
3613,
265,
62,
18,
60,
198,
198,
23160,
62,
16,
796,
685,
452,
13,
20274,
58,
16,
4083,
8367,
329,
21628,
287,
1709,
2246,
62,
738,
62,
16,
60,
198,
549,
62,
16,
796,
685,
452,
13,
20274,
58,
17,
4083,
8367,
329,
21628,
287,
1709,
2246,
62,
738,
62,
16,
60,
198,
198,
17201,
62,
16,
796,
685,
452,
13,
20274,
58,
16,
4083,
24588,
329,
21628,
287,
1709,
2246,
62,
738,
62,
16,
60,
198,
84,
2676,
62,
16,
796,
685,
452,
13,
20274,
58,
17,
4083,
24588,
329,
21628,
287,
1709,
2246,
62,
738,
62,
16,
60,
198,
198,
7568,
796,
6060,
19778,
7,
22355,
796,
3613,
265,
62,
16,
11,
2793,
796,
18360,
62,
16,
11,
6727,
796,
20967,
62,
16,
11,
4187,
796,
4187,
62,
16,
11,
334,
2676,
796,
334,
2676,
62,
16,
8,
198,
7568,
13,
21037,
796,
6330,
7,
7568,
13,
21037,
11,
2147,
5218,
4814,
8,
198,
7568,
13,
45828,
796,
6330,
7,
7568,
13,
45828,
11,
2147,
5218,
4814,
8,
198,
7902,
53,
13,
13564,
7,
1911,
14,
73,
43640,
14,
1416,
77,
16,
62,
10414,
62,
3903,
13,
40664,
1600,
47764,
8,
198,
198,
9414,
2246,
62,
16,
796,
985,
62,
1416,
77,
16,
12195,
21928,
265,
62,
16,
11,
1058,
9414,
2246,
8,
198,
29487,
7,
14323,
62,
1416,
77,
16,
11,
905,
62,
1326,
5015,
902,
28,
9562,
11,
410,
945,
41888,
25,
9414,
2246,
12962,
198,
1416,
1436,
0,
7,
7890,
62,
1416,
77,
58,
25,
1416,
77,
16,
4083,
83,
11,
1366,
62,
1416,
77,
58,
25,
1416,
77,
16,
4083,
1326,
5015,
434,
11,
331,
18224,
28,
7890,
62,
1416,
77,
58,
25,
1416,
77,
16,
7131,
0,
553,
1676,
65,
13,
82,
13495,
33116,
6167,
796,
366,
47384,
902,
4943,
198,
29487,
0,
7,
21928,
265,
62,
16,
11,
18360,
62,
16,
11,
6070,
9521,
796,
220,
20967,
62,
16,
11,
6070,
26591,
796,
657,
13,
2327,
11,
269,
796,
352,
11,
6167,
796,
366,
18546,
1704,
4097,
4943,
198,
21928,
5647,
7,
1911,
14,
73,
43640,
14,
10414,
62,
3903,
13,
11134,
4943,
198,
198,
23160,
62,
17,
796,
685,
452,
13,
20274,
58,
16,
4083,
8367,
329,
21628,
287,
1709,
2246,
62,
738,
62,
17,
60,
198,
549,
62,
17,
796,
685,
452,
13,
20274,
58,
17,
4083,
8367,
329,
21628,
287,
1709,
2246,
62,
738,
62,
17,
60,
198,
9414,
2246,
62,
17,
796,
985,
62,
1416,
77,
17,
12195,
21928,
265,
62,
17,
11,
1058,
9414,
2246,
8,
198,
29487,
7,
14323,
62,
1416,
77,
17,
11,
905,
62,
1326,
5015,
902,
28,
9562,
11,
29092,
796,
357,
9414,
2246,
62,
17,
12,
23160,
62,
16,
11,
549,
62,
17,
12,
9414,
2246,
62,
17,
828,
277,
66,
28,
25,
43745,
11,
24685,
28,
15,
13,
22,
8,
198,
198,
23160,
62,
18,
796,
685,
452,
13,
20274,
58,
16,
4083,
8367,
329,
21628,
287,
1709,
2246,
62,
738,
62,
18,
60,
198,
549,
62,
18,
796,
685,
452,
13,
20274,
58,
17,
4083,
8367,
329,
21628,
287,
1709,
2246,
62,
738,
62,
18,
60,
198,
9414,
2246,
62,
18,
796,
985,
62,
1416,
77,
18,
12195,
21928,
265,
62,
18,
11,
1058,
9414,
2246,
8,
198,
29487,
7,
14323,
62,
1416,
77,
18,
11,
905,
62,
1326,
5015,
902,
28,
9562,
11,
29092,
796,
357,
9414,
2246,
62,
18,
12,
23160,
62,
18,
11,
549,
62,
18,
12,
9414,
2246,
62,
18,
828,
277,
66,
28,
25,
43745,
11,
24685,
28,
15,
13,
22,
8,
198,
198,
21017,
3254,
24765,
4097,
198,
198,
83,
62,
1416,
77,
16,
796,
1366,
62,
1416,
77,
58,
25,
1416,
77,
16,
4083,
83,
198,
82,
13495,
62,
1416,
77,
16,
796,
1366,
62,
1416,
77,
58,
25,
1416,
77,
16,
7131,
0,
553,
1676,
65,
13,
82,
13495,
8973,
198,
14323,
62,
1416,
77,
16,
796,
985,
7,
79,
13,
1416,
268,
13010,
58,
25,
1416,
77,
16,
11208,
10007,
62,
929,
67,
28,
79,
62,
40085,
62,
16,
11,
823,
83,
349,
28,
16,
68,
12,
21,
11,
16552,
349,
28,
16,
68,
12,
23,
8,
198,
9414,
2246,
62,
1416,
77,
16,
796,
985,
62,
1416,
77,
16,
12195,
83,
62,
1416,
77,
16,
11,
1058,
9414,
2246,
8,
198,
198,
8818,
4938,
62,
26801,
16,
7,
37266,
11,
1312,
8,
198,
220,
288,
16,
796,
938,
7,
37266,
8,
198,
220,
4808,
37266,
796,
685,
21999,
5218,
279,
85,
329,
357,
21999,
11,
79,
85,
8,
287,
19974,
7,
79,
62,
14933,
58,
25,
1416,
77,
16,
4357,
37266,
58,
16,
25,
437,
12,
16,
12962,
60,
628,
220,
985,
62,
411,
796,
985,
7,
79,
13,
1416,
268,
13010,
58,
25,
1416,
77,
16,
11208,
10007,
62,
929,
67,
28,
62,
37266,
11,
823,
83,
349,
28,
16,
68,
12,
21,
11,
16552,
349,
28,
16,
68,
12,
23,
8,
198,
220,
288,
16,
62,
14323,
796,
985,
62,
411,
7,
83,
62,
1416,
77,
16,
58,
72,
12962,
58,
25,
9414,
2246,
60,
198,
220,
1441,
2994,
7,
14323,
62,
411,
11,
985,
62,
411,
13,
1416,
39055,
13,
1326,
5015,
902,
8,
1343,
357,
67,
16,
532,
288,
16,
62,
14323,
8,
61,
17,
29006,
82,
13495,
62,
1416,
77,
16,
58,
72,
12962,
61,
17,
532,
2994,
62,
2860,
58,
25,
1416,
77,
16,
60,
198,
437,
198,
198,
12102,
62,
16,
796,
17635,
198,
1640,
1312,
287,
1123,
9630,
7,
83,
62,
1416,
77,
16,
8,
220,
198,
35235,
7203,
27131,
803,
14514,
329,
29568,
83,
62,
1416,
77,
16,
58,
72,
12962,
640,
4122,
4943,
198,
14689,
0,
7,
12102,
62,
16,
11,
220,
198,
220,
651,
62,
3849,
2100,
7,
198,
220,
220,
220,
685,
12957,
12195,
79,
62,
40085,
62,
16,
1776,
1709,
2246,
62,
1416,
77,
16,
58,
72,
60,
4357,
198,
220,
220,
220,
642,
11,
198,
220,
220,
220,
279,
3784,
12102,
62,
26801,
16,
7,
79,
11,
72,
828,
198,
220,
220,
220,
1058,
34,
22707,
62,
11651,
62,
47924,
11,
198,
220,
220,
220,
262,
8326,
62,
65,
3733,
796,
6070,
19510,
16,
68,
12,
23,
11,
16,
68,
23,
828,
4129,
7,
79,
62,
14933,
58,
25,
1416,
77,
16,
12962,
10,
16,
828,
198,
220,
220,
220,
9367,
62,
65,
3733,
16193,
16,
68,
12,
22,
11,
16,
68,
22,
828,
220,
198,
220,
220,
220,
9367,
62,
83,
349,
28,
16,
68,
12,
20,
11,
198,
220,
220,
220,
5046,
796,
220,
6070,
7,
25,
6404,
11,
4129,
7,
79,
62,
14933,
58,
25,
1416,
77,
16,
12962,
10,
16,
828,
198,
220,
220,
220,
2994,
62,
22213,
796,
2994,
62,
1416,
77,
16,
7,
79,
62,
40085,
62,
16,
8,
1343,
33166,
62,
5715,
8,
198,
220,
1267,
198,
437,
198,
198,
23160,
62,
16,
796,
685,
452,
13,
20274,
58,
16,
4083,
8367,
329,
21628,
287,
4938,
62,
16,
60,
198,
549,
62,
16,
796,
685,
452,
13,
20274,
58,
17,
4083,
8367,
329,
21628,
287,
4938,
62,
16,
60,
198,
198,
17201,
62,
16,
796,
685,
452,
13,
20274,
58,
16,
4083,
24588,
329,
21628,
287,
4938,
62,
16,
60,
198,
84,
2676,
62,
16,
796,
685,
452,
13,
20274,
58,
17,
4083,
24588,
329,
21628,
287,
4938,
62,
16,
60,
198,
198,
7568,
796,
6060,
19778,
7,
22355,
796,
256,
62,
1416,
77,
16,
11,
2793,
796,
18360,
62,
16,
11,
6727,
796,
20967,
62,
16,
11,
4187,
796,
4187,
62,
16,
11,
334,
2676,
796,
334,
2676,
62,
16,
8,
198,
7568,
13,
21037,
796,
6330,
7,
7568,
13,
21037,
11,
2147,
5218,
4814,
8,
198,
7568,
13,
45828,
796,
6330,
7,
7568,
13,
45828,
11,
2147,
5218,
4814,
8,
198,
7902,
53,
13,
13564,
7,
1911,
14,
73,
43640,
14,
1416,
77,
16,
62,
12102,
62,
65,
504,
13,
40664,
1600,
47764,
8,
198,
198,
23160,
62,
16,
58,
16,
60,
796,
657,
13,
15,
198,
23160,
62,
16,
58,
437,
12,
19,
25,
437,
60,
764,
28,
657,
13,
15,
198,
29487,
7,
14323,
62,
1416,
77,
16,
11,
905,
62,
1326,
5015,
902,
28,
9562,
11,
410,
945,
41888,
25,
9414,
2246,
12962,
198,
1416,
1436,
0,
7,
7890,
62,
1416,
77,
58,
25,
1416,
77,
16,
4083,
83,
11,
1366,
62,
1416,
77,
58,
25,
1416,
77,
16,
4083,
1326,
5015,
434,
11,
331,
18224,
28,
7890,
62,
1416,
77,
58,
25,
1416,
77,
16,
7131,
0,
553,
1676,
65,
13,
82,
13495,
33116,
6167,
796,
366,
47384,
902,
4943,
198,
29487,
0,
7,
83,
62,
1416,
77,
16,
11,
18360,
62,
16,
11,
6070,
9521,
796,
220,
20967,
62,
16,
11,
6070,
26591,
796,
657,
13,
2327,
11,
269,
796,
352,
11,
6167,
796,
366,
7762,
24765,
4097,
4943,
198,
21928,
5647,
7,
1911,
14,
73,
43640,
14,
12102,
62,
3903,
13,
11134,
4943
] | 2.155268 | 4,167 |
export TabularRandomPolicy
"""
TabularRandomPolicy(prob::Array{Float64, 2})
`prob` describes the distribution of actions for each state.
"""
struct TabularRandomPolicy <: AbstractPolicy
prob::Array{Float64,2}
end
(π::TabularRandomPolicy)(s) = sample(Weights(π.prob[s, :]))
(π::TabularRandomPolicy)(obs::Observation) = π(get_state(obs))
get_prob(π::TabularRandomPolicy, s) = @view π.prob[s, :]
get_prob(π::TabularRandomPolicy, s, a) = π.prob[s, a] | [
39344,
16904,
934,
29531,
36727,
198,
198,
37811,
198,
220,
220,
220,
16904,
934,
29531,
36727,
7,
1676,
65,
3712,
19182,
90,
43879,
2414,
11,
362,
30072,
198,
198,
63,
1676,
65,
63,
8477,
262,
6082,
286,
4028,
329,
1123,
1181,
13,
198,
37811,
198,
7249,
16904,
934,
29531,
36727,
1279,
25,
27741,
36727,
198,
220,
220,
220,
1861,
3712,
19182,
90,
43879,
2414,
11,
17,
92,
198,
437,
198,
198,
7,
46582,
3712,
33349,
934,
29531,
36727,
5769,
82,
8,
796,
6291,
7,
1135,
2337,
7,
46582,
13,
1676,
65,
58,
82,
11,
1058,
60,
4008,
198,
7,
46582,
3712,
33349,
934,
29531,
36727,
5769,
8158,
3712,
31310,
13208,
8,
796,
18074,
222,
7,
1136,
62,
5219,
7,
8158,
4008,
198,
198,
1136,
62,
1676,
65,
7,
46582,
3712,
33349,
934,
29531,
36727,
11,
264,
8,
796,
2488,
1177,
18074,
222,
13,
1676,
65,
58,
82,
11,
1058,
60,
198,
1136,
62,
1676,
65,
7,
46582,
3712,
33349,
934,
29531,
36727,
11,
264,
11,
257,
8,
796,
18074,
222,
13,
1676,
65,
58,
82,
11,
257,
60
] | 2.573034 | 178 |
export elu, relu, selu, sigm, invx
using AutoGrad: AutoGrad, @primitive
"""
elu(x)
Return `(x > 0 ? x : exp(x)-1)`.
Reference: Fast and Accurate Deep Network Learning by Exponential Linear Units (ELUs) (https://arxiv.org/abs/1511.07289).
"""
elu(x::T) where T = (x >= 0 ? x : exp(x)-T(1))
eluback(dy::T,y::T) where T = (y >= 0 ? dy : dy * (T(1)+y))
@primitive elu(x),dy,y eluback.(dy,y)
@primitive eluback(dy,y),ddx (ddx.*((y.>=0).+(y.<0).*(y.+1))) (ddx.*dy.*(y.<0))
"""
relu(x)
Return `max(0,x)`.
References:
* [Nair and Hinton, 2010](https://icml.cc/Conferences/2010/abstracts.html#432). Rectified Linear Units Improve Restricted Boltzmann Machines. ICML.
* [Glorot, Bordes and Bengio, 2011](http://proceedings.mlr.press/v15/glorot11a). Deep Sparse Rectifier Neural Networks. AISTATS.
"""
relu(x::T) where T = max(x,T(0))
reluback(dy::T,y::T) where T = (y>0 ? dy : T(0))
@primitive relu(x),dy,y reluback.(dy,y)
@primitive reluback(dy,y),ddx (ddx.*(y.>0)) nothing
"""
selu(x)
Return `λ01 * (x > 0 ? x : α01 * (exp(x)-1))` where `λ01=1.0507009873554805` and `α01=1.6732632423543778`.
Reference: Self-Normalizing Neural Networks (https://arxiv.org/abs/1706.02515).
"""
selu(x::T) where T = (x >= 0 ? T(λ01)*x : T(λα01)*(exp(x)-T(1)))
seluback(dy::T,y::T) where T = (y >= 0 ? dy * T(λ01) : dy * (y + T(λα01)))
@primitive selu(x),dy,y seluback.(dy,y)
@primitive seluback(dy,y),ddx (T=eltype(y); ddx.*((y.>=0).*T(λ01).+(y.<0).*(y.+T(λα01)))) (ddx.*dy.*(y.<0))
const λ01 = 1.0507009873554805 # (1-erfc(1/sqrt(2))*sqrt(exp(1)))*sqrt(2pi)*(2*erfc(sqrt(2))*exp(2)+pi*erfc(1/sqrt(2))^2*exp(1)-2*(2+pi)*erfc(1/sqrt(2))*sqrt(exp(1))+pi+2)^(-0.5)
const α01 = 1.6732632423543778 # -sqrt(2/pi)/(erfc(1/sqrt(2))*exp(1/2)-1)
const λα01 = 1.7580993408473773 # λ01 * α01
"""
sigm(x)
Return `1/(1+exp(-x))`.
Reference: Numerically stable sigm implementation from http://timvieira.github.io/blog/post/2014/02/11/exp-normalize-trick.
"""
sigm(x::T) where T = (x >= 0 ? T(1)/(T(1)+exp(-x)) : (z=exp(x); z/(T(1)+z)))
sigmback(dy::T,y::T) where T = (dy*y*(T(1)-y))
@primitive sigm(x),dy,y sigmback.(dy,y)
@primitive sigmback(dy,y),ddx ddx.*y.*(1 .- y) ddx.*dy.*(1 .- 2 .* y)
function invx(x)
@warn "invx() is deprecated, please use 1/x instead" maxlog=1
1/x
end
| [
39344,
1288,
84,
11,
823,
84,
11,
384,
2290,
11,
264,
17225,
11,
800,
87,
198,
3500,
11160,
42731,
25,
11160,
42731,
11,
2488,
19795,
1800,
628,
198,
37811,
198,
220,
220,
220,
1288,
84,
7,
87,
8,
198,
198,
13615,
4600,
7,
87,
1875,
657,
5633,
2124,
1058,
1033,
7,
87,
13219,
16,
8,
44646,
198,
198,
26687,
25,
12549,
290,
6366,
15537,
10766,
7311,
18252,
416,
5518,
35470,
44800,
27719,
357,
3698,
5842,
8,
357,
5450,
1378,
283,
87,
452,
13,
2398,
14,
8937,
14,
1314,
1157,
13,
2998,
27693,
737,
198,
37811,
198,
417,
84,
7,
87,
3712,
51,
8,
810,
309,
796,
357,
87,
18189,
657,
5633,
2124,
1058,
1033,
7,
87,
13219,
51,
7,
16,
4008,
198,
417,
549,
441,
7,
9892,
3712,
51,
11,
88,
3712,
51,
8,
810,
309,
796,
357,
88,
18189,
657,
5633,
20268,
1058,
20268,
1635,
357,
51,
7,
16,
47762,
88,
4008,
198,
31,
19795,
1800,
1288,
84,
7,
87,
828,
9892,
11,
88,
1288,
549,
441,
12195,
9892,
11,
88,
8,
198,
31,
19795,
1800,
1288,
549,
441,
7,
9892,
11,
88,
828,
1860,
87,
220,
357,
1860,
87,
15885,
19510,
88,
13,
29,
28,
15,
737,
33747,
88,
29847,
15,
737,
9,
7,
88,
13,
10,
16,
22305,
220,
357,
1860,
87,
15885,
9892,
15885,
7,
88,
29847,
15,
4008,
628,
198,
37811,
198,
220,
220,
220,
823,
84,
7,
87,
8,
198,
13615,
4600,
9806,
7,
15,
11,
87,
8,
44646,
198,
198,
19927,
25,
220,
198,
9,
685,
45,
958,
290,
367,
2371,
11,
3050,
16151,
5450,
1378,
291,
4029,
13,
535,
14,
18546,
4972,
14,
10333,
14,
397,
8709,
82,
13,
6494,
2,
45331,
737,
48599,
1431,
44800,
27719,
20580,
8324,
20941,
21764,
89,
9038,
31182,
13,
12460,
5805,
13,
198,
9,
685,
38,
4685,
313,
11,
347,
35770,
290,
14964,
952,
11,
2813,
16151,
4023,
1378,
1676,
2707,
654,
13,
4029,
81,
13,
8439,
14,
85,
1314,
14,
70,
4685,
313,
1157,
64,
737,
10766,
1338,
17208,
48599,
7483,
47986,
27862,
13,
317,
8808,
33586,
13,
198,
37811,
198,
260,
2290,
7,
87,
3712,
51,
8,
810,
309,
796,
3509,
7,
87,
11,
51,
7,
15,
4008,
198,
2411,
549,
441,
7,
9892,
3712,
51,
11,
88,
3712,
51,
8,
810,
309,
796,
357,
88,
29,
15,
5633,
20268,
1058,
309,
7,
15,
4008,
198,
31,
19795,
1800,
823,
84,
7,
87,
828,
9892,
11,
88,
823,
549,
441,
12195,
9892,
11,
88,
8,
198,
31,
19795,
1800,
823,
549,
441,
7,
9892,
11,
88,
828,
1860,
87,
220,
357,
1860,
87,
15885,
7,
88,
13,
29,
15,
4008,
220,
2147,
628,
198,
37811,
198,
220,
220,
220,
384,
2290,
7,
87,
8,
198,
198,
13615,
4600,
39377,
486,
1635,
357,
87,
1875,
657,
5633,
2124,
1058,
26367,
486,
1635,
357,
11201,
7,
87,
13219,
16,
4008,
63,
810,
4600,
39377,
486,
28,
16,
13,
28669,
9879,
44183,
2327,
4051,
28256,
63,
290,
4600,
17394,
486,
28,
16,
13,
45758,
29558,
1731,
1954,
4051,
2718,
3695,
44646,
198,
198,
26687,
25,
12189,
12,
26447,
2890,
47986,
27862,
357,
5450,
1378,
283,
87,
452,
13,
2398,
14,
8937,
14,
1558,
3312,
13,
36629,
1314,
737,
198,
37811,
198,
741,
84,
7,
87,
3712,
51,
8,
810,
309,
796,
357,
87,
18189,
657,
5633,
309,
7,
39377,
486,
27493,
87,
1058,
309,
7,
39377,
17394,
486,
27493,
7,
11201,
7,
87,
13219,
51,
7,
16,
22305,
198,
741,
549,
441,
7,
9892,
3712,
51,
11,
88,
3712,
51,
8,
810,
309,
796,
357,
88,
18189,
657,
5633,
20268,
1635,
309,
7,
39377,
486,
8,
1058,
20268,
1635,
357,
88,
1343,
309,
7,
39377,
17394,
486,
22305,
198,
31,
19795,
1800,
384,
2290,
7,
87,
828,
9892,
11,
88,
384,
75,
549,
441,
12195,
9892,
11,
88,
8,
198,
31,
19795,
1800,
384,
75,
549,
441,
7,
9892,
11,
88,
828,
1860,
87,
220,
357,
51,
28,
417,
4906,
7,
88,
1776,
288,
34350,
15885,
19510,
88,
13,
29,
28,
15,
737,
9,
51,
7,
39377,
486,
737,
33747,
88,
29847,
15,
737,
9,
7,
88,
13,
10,
51,
7,
39377,
17394,
486,
35514,
220,
357,
1860,
87,
15885,
9892,
15885,
7,
88,
29847,
15,
4008,
198,
9979,
7377,
119,
486,
796,
352,
13,
28669,
9879,
44183,
2327,
4051,
28256,
220,
1303,
357,
16,
12,
263,
16072,
7,
16,
14,
31166,
17034,
7,
17,
4008,
9,
31166,
17034,
7,
11201,
7,
16,
22305,
9,
31166,
17034,
7,
17,
14415,
27493,
7,
17,
9,
263,
16072,
7,
31166,
17034,
7,
17,
4008,
9,
11201,
7,
17,
47762,
14415,
9,
263,
16072,
7,
16,
14,
31166,
17034,
7,
17,
4008,
61,
17,
9,
11201,
7,
16,
13219,
17,
9,
7,
17,
10,
14415,
27493,
263,
16072,
7,
16,
14,
31166,
17034,
7,
17,
4008,
9,
31166,
17034,
7,
11201,
7,
16,
4008,
10,
14415,
10,
17,
8,
61,
32590,
15,
13,
20,
8,
198,
9979,
26367,
486,
796,
352,
13,
45758,
29558,
1731,
1954,
4051,
2718,
3695,
220,
1303,
532,
31166,
17034,
7,
17,
14,
14415,
20679,
7,
263,
16072,
7,
16,
14,
31166,
17034,
7,
17,
4008,
9,
11201,
7,
16,
14,
17,
13219,
16,
8,
198,
9979,
7377,
119,
17394,
486,
796,
352,
13,
2425,
1795,
2079,
23601,
23,
2857,
2718,
4790,
1303,
7377,
119,
486,
1635,
26367,
486,
628,
198,
37811,
198,
220,
220,
220,
264,
17225,
7,
87,
8,
198,
198,
13615,
4600,
16,
29006,
16,
10,
11201,
32590,
87,
4008,
44646,
198,
198,
26687,
25,
399,
6975,
1146,
8245,
264,
17225,
7822,
422,
2638,
1378,
16514,
85,
494,
8704,
13,
12567,
13,
952,
14,
14036,
14,
7353,
14,
4967,
14,
2999,
14,
1157,
14,
11201,
12,
11265,
1096,
12,
2213,
624,
13,
198,
37811,
198,
82,
17225,
7,
87,
3712,
51,
8,
810,
309,
796,
357,
87,
18189,
657,
5633,
309,
7,
16,
20679,
7,
51,
7,
16,
47762,
11201,
32590,
87,
4008,
1058,
357,
89,
28,
11201,
7,
87,
1776,
1976,
29006,
51,
7,
16,
47762,
89,
22305,
198,
82,
17225,
1891,
7,
9892,
3712,
51,
11,
88,
3712,
51,
8,
810,
309,
796,
357,
9892,
9,
88,
9,
7,
51,
7,
16,
13219,
88,
4008,
198,
31,
19795,
1800,
264,
17225,
7,
87,
828,
9892,
11,
88,
220,
264,
17225,
1891,
12195,
9892,
11,
88,
8,
198,
31,
19795,
1800,
264,
17225,
1891,
7,
9892,
11,
88,
828,
1860,
87,
220,
288,
34350,
15885,
88,
15885,
7,
16,
764,
12,
331,
8,
220,
288,
34350,
15885,
9892,
15885,
7,
16,
764,
12,
362,
764,
9,
331,
8,
198,
198,
8818,
800,
87,
7,
87,
8,
198,
220,
220,
220,
2488,
40539,
366,
16340,
87,
3419,
318,
39224,
11,
3387,
779,
352,
14,
87,
2427,
1,
3509,
6404,
28,
16,
198,
220,
220,
220,
352,
14,
87,
198,
437,
198
] | 2.030142 | 1,128 |
function extract_node_list!(node::XMLElement, nodeArray::Array{XMLElement,1}, label::String)
# get kids of node -
list_of_children = collect(child_elements(node))
for child_node in list_of_children
if (name(child_node) == label)
push!(nodeArray, child_node)
else
extract_node_list!(child_node, nodeArray, label)
end
end
end
function extract_reactant_list(node::XMLElement)
species_array = Array{XMLElement,1}()
list_of_reactants_node = Array{XMLElement,1}()
# get list of reactants -
extract_node_list!(node,list_of_reactants_node,"listOfReactants")
# get the species ref kids -
list_of_children = collect(child_elements(list_of_reactants_node[1])) # we *should* have only one of these -
for child_node in list_of_children
push!(species_array,child_node)
end
return species_array
end
function extract_product_list(node::XMLElement)
species_array = Array{XMLElement,1}()
list_of_products_node = Array{XMLElement,1}()
# get list of reactants -
extract_node_list!(node,list_of_products_node,"listOfProducts")
# get the species ref kids -
list_of_children = collect(child_elements(list_of_products_node[1])) # we *should* have only one of these -
for child_node in list_of_children
push!(species_array,child_node)
end
return species_array
end
function build_metabolic_reaction_object_array(tree_root::XMLElement)::Array{VLMetabolicReaction,1}
# initialize -
reaction_object_array = Array{VLMetabolicReaction,1}()
tmp_reaction_array = Array{XMLElement,1}()
# extract the list of reaction objects -
extract_node_list!(tree_root, tmp_reaction_array, "reaction")
for xml_reaction_object in tmp_reaction_array
# build new reaction object -
robject = VLMetabolicReaction()
# get data -
rname = attribute(xml_reaction_object,"id")
rev_flag = attribute(xml_reaction_object,"reversible")
# build reactions phrases -
list_of_reactants = extract_reactant_list(xml_reaction_object)
list_of_products = extract_product_list(xml_reaction_object)
# left phase -
left_phrase = ""
for species_ref_tag in list_of_reactants
# species id and stoichiometry -
species = attribute(species_ref_tag,"species")
stcoeff = attribute(species_ref_tag, "stoichiometry")
left_phrase *= "$(stcoeff)*$(species)+"
end
left_phrase = left_phrase[1:end-1] # cutoff the trailing +
# right phrase -
right_phrase = ""
for species_ref_tag in list_of_products
# species id and stoichiometry -
species = attribute(species_ref_tag,"species")
stcoeff = attribute(species_ref_tag, "stoichiometry")
right_phrase *= "$(stcoeff)*$(species)+"
end
right_phrase = right_phrase[1:end-1] # cuttoff the trailing +
# populate the reaction object -
robject.reaction_name = rname
robject.ec_number = "[]"
robject.reversible = rev_flag
robject.left_phrase = left_phrase
robject.right_phrase = right_phrase
# cache -
push!(reaction_object_array, robject)
end
# return -
return reaction_object_array
end
function build_txtl_program_component(tree_root::XMLElement, filename::String)::VLProgramComponent
# initialize -
program_component = VLProgramComponent()
# load the header text for TXTL -
path_to_impl = "$(path_to_package)/distribution/julia/include/TXTL-Header-Section.txt"
buffer = include_function(path_to_impl)
# collapse -
flat_buffer = ""
[flat_buffer *= line for line in buffer]
# add data to program_component -
program_component.filename = filename;
program_component.buffer = flat_buffer
program_component.type = :buffer
# return -
return program_component
end
function build_grn_program_component(tree_root::XMLElement, filename::String)::VLProgramComponent
# initialize -
program_component = VLProgramComponent()
# load the header text for TXTL -
path_to_impl = "$(path_to_package)/distribution/julia/include/GRN-Header-Section.txt"
buffer = include_function(path_to_impl)
# collapse -
flat_buffer = ""
[flat_buffer *= line for line in buffer]
# add data to program_component -
program_component.filename = filename;
program_component.buffer = flat_buffer
program_component.type = :buffer
# return -
return program_component
end
function build_global_header_program_component(tree_root::XMLElement, filename::String)::VLProgramComponent
# initialize -
program_component = VLProgramComponent()
# load the header text for TXTL -
path_to_impl = "$(path_to_package)/distribution/julia/include/Global-Header-Section.txt"
buffer = include_function(path_to_impl)
# collapse -
flat_buffer = ""
[flat_buffer *= line for line in buffer]
# add data to program_component -
program_component.filename = filename;
program_component.buffer = flat_buffer
program_component.type = :buffer
# return -
return program_component
end
function build_metabolism_program_component(tree_root::XMLElement, filename::String)::VLProgramComponent
# initialize -
buffer = Array{String,1}()
program_component = VLProgramComponent()
# header information -
+(buffer, "// ***************************************************************************** //\n")
+(buffer, "#METABOLISM::START\n")
+(buffer, "// Metabolism record format:\n")
+(buffer, "// reaction_name (unique), [{; delimited set of ec numbers | []}],reactant_string,product_string,reversible\n")
+(buffer,"//\n")
+(buffer, "// Rules:\n");
+(buffer, "// The reaction_name field is unique, and metabolite symbols can not have special chars or spaces\n")
+(buffer, "//\n")
+(buffer, "// Example:\n")
+(buffer, "// R_A_syn_2,[6.3.4.13],M_atp_c+M_5pbdra+M_gly_L_c,M_adp_c+M_pi_c+M_gar_c,false\n")
+(buffer, "//\n")
+(buffer, "// Stochiometric coefficients are pre-pended to metabolite symbol, for example:\n")
+(buffer, "// R_adhE,[1.2.1.10; 1.1.1.1],M_accoa_c+2*M_h_c+2*M_nadh_c,M_coa_c+M_etoh_c+2*M_nad_c,true\n")
+(buffer, "\n")
# build the reaction array of objects -
reaction_object_array = build_metabolic_reaction_object_array(tree_root)
tmp_string = ""
for reaction_object::VLMetabolicReaction in reaction_object_array
# get data -
reaction_name = reaction_object.reaction_name
ec_number = reaction_object.ec_number
left_phrase = reaction_object.left_phrase
right_phrase = reaction_object.right_phrase
reversible_flag = reaction_object.reversible
# build a reaction string -
tmp_string = "$(reaction_name),$(ec_number),$(left_phrase),$(right_phrase),$(reversible_flag)\n"
# push onto the buffer -
+(buffer, tmp_string)
# clear -
tmp_string = []
end
# close the section -
+(buffer, "\n")
+(buffer, "#METABOLISM::STOP\n")
+(buffer, "// ***************************************************************************** //\n")
# collapse -
flat_buffer = ""
[flat_buffer *= line for line in buffer]
# add data to program_component -
program_component.filename = filename;
program_component.buffer = flat_buffer
program_component.type = :buffer
# return -
return program_component
end | [
8818,
7925,
62,
17440,
62,
4868,
0,
7,
17440,
3712,
37643,
2538,
1732,
11,
10139,
19182,
3712,
19182,
90,
37643,
2538,
1732,
11,
16,
5512,
6167,
3712,
10100,
8,
628,
220,
220,
220,
1303,
651,
3988,
286,
10139,
532,
198,
220,
220,
220,
1351,
62,
1659,
62,
17197,
796,
2824,
7,
9410,
62,
68,
3639,
7,
17440,
4008,
198,
220,
220,
220,
329,
1200,
62,
17440,
287,
1351,
62,
1659,
62,
17197,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
611,
357,
3672,
7,
9410,
62,
17440,
8,
6624,
6167,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
17440,
19182,
11,
1200,
62,
17440,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7925,
62,
17440,
62,
4868,
0,
7,
9410,
62,
17440,
11,
10139,
19182,
11,
6167,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
7925,
62,
45018,
415,
62,
4868,
7,
17440,
3712,
37643,
2538,
1732,
8,
628,
220,
220,
220,
4693,
62,
18747,
796,
15690,
90,
37643,
2538,
1732,
11,
16,
92,
3419,
198,
220,
220,
220,
1351,
62,
1659,
62,
45018,
1187,
62,
17440,
796,
15690,
90,
37643,
2538,
1732,
11,
16,
92,
3419,
628,
220,
220,
220,
1303,
651,
1351,
286,
6324,
1187,
532,
198,
220,
220,
220,
7925,
62,
17440,
62,
4868,
0,
7,
17440,
11,
4868,
62,
1659,
62,
45018,
1187,
62,
17440,
553,
4868,
5189,
3041,
529,
1187,
4943,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
651,
262,
4693,
1006,
3988,
532,
198,
220,
220,
220,
1351,
62,
1659,
62,
17197,
796,
2824,
7,
9410,
62,
68,
3639,
7,
4868,
62,
1659,
62,
45018,
1187,
62,
17440,
58,
16,
60,
4008,
1303,
356,
1635,
21754,
9,
423,
691,
530,
286,
777,
532,
198,
220,
220,
220,
329,
1200,
62,
17440,
287,
1351,
62,
1659,
62,
17197,
198,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
35448,
62,
18747,
11,
9410,
62,
17440,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1441,
4693,
62,
18747,
198,
437,
198,
198,
8818,
7925,
62,
11167,
62,
4868,
7,
17440,
3712,
37643,
2538,
1732,
8,
628,
220,
220,
220,
4693,
62,
18747,
796,
15690,
90,
37643,
2538,
1732,
11,
16,
92,
3419,
198,
220,
220,
220,
1351,
62,
1659,
62,
29498,
62,
17440,
796,
15690,
90,
37643,
2538,
1732,
11,
16,
92,
3419,
628,
220,
220,
220,
1303,
651,
1351,
286,
6324,
1187,
532,
198,
220,
220,
220,
7925,
62,
17440,
62,
4868,
0,
7,
17440,
11,
4868,
62,
1659,
62,
29498,
62,
17440,
553,
4868,
5189,
48650,
4943,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
651,
262,
4693,
1006,
3988,
532,
198,
220,
220,
220,
1351,
62,
1659,
62,
17197,
796,
2824,
7,
9410,
62,
68,
3639,
7,
4868,
62,
1659,
62,
29498,
62,
17440,
58,
16,
60,
4008,
1303,
356,
1635,
21754,
9,
423,
691,
530,
286,
777,
532,
198,
220,
220,
220,
329,
1200,
62,
17440,
287,
1351,
62,
1659,
62,
17197,
198,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
35448,
62,
18747,
11,
9410,
62,
17440,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1441,
4693,
62,
18747,
198,
437,
198,
198,
8818,
1382,
62,
4164,
29304,
62,
260,
2673,
62,
15252,
62,
18747,
7,
21048,
62,
15763,
3712,
37643,
2538,
1732,
2599,
25,
19182,
90,
47468,
9171,
29304,
3041,
2673,
11,
16,
92,
628,
220,
220,
220,
1303,
41216,
532,
198,
220,
220,
220,
6317,
62,
15252,
62,
18747,
796,
15690,
90,
47468,
9171,
29304,
3041,
2673,
11,
16,
92,
3419,
198,
220,
220,
220,
45218,
62,
260,
2673,
62,
18747,
796,
15690,
90,
37643,
2538,
1732,
11,
16,
92,
3419,
628,
220,
220,
220,
1303,
7925,
262,
1351,
286,
6317,
5563,
532,
198,
220,
220,
220,
7925,
62,
17440,
62,
4868,
0,
7,
21048,
62,
15763,
11,
45218,
62,
260,
2673,
62,
18747,
11,
366,
260,
2673,
4943,
198,
220,
220,
220,
329,
35555,
62,
260,
2673,
62,
15252,
287,
45218,
62,
260,
2673,
62,
18747,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
1382,
649,
6317,
2134,
532,
198,
220,
220,
220,
220,
220,
220,
220,
3857,
752,
796,
569,
43,
9171,
29304,
3041,
2673,
3419,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
651,
1366,
532,
198,
220,
220,
220,
220,
220,
220,
220,
374,
3672,
796,
11688,
7,
19875,
62,
260,
2673,
62,
15252,
553,
312,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
2710,
62,
32109,
796,
11688,
7,
19875,
62,
260,
2673,
62,
15252,
553,
260,
37393,
4943,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
1382,
12737,
20144,
532,
198,
220,
220,
220,
220,
220,
220,
220,
1351,
62,
1659,
62,
45018,
1187,
796,
7925,
62,
45018,
415,
62,
4868,
7,
19875,
62,
260,
2673,
62,
15252,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1351,
62,
1659,
62,
29498,
796,
7925,
62,
11167,
62,
4868,
7,
19875,
62,
260,
2673,
62,
15252,
8,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
1364,
7108,
532,
198,
220,
220,
220,
220,
220,
220,
220,
1364,
62,
34675,
796,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
329,
4693,
62,
5420,
62,
12985,
287,
1351,
62,
1659,
62,
45018,
1187,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
4693,
4686,
290,
3995,
16590,
15748,
532,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4693,
796,
11688,
7,
35448,
62,
5420,
62,
12985,
553,
35448,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
336,
1073,
14822,
796,
11688,
7,
35448,
62,
5420,
62,
12985,
11,
366,
301,
78,
16590,
15748,
4943,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1364,
62,
34675,
1635,
28,
17971,
7,
301,
1073,
14822,
27493,
3,
7,
35448,
47762,
1,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
1364,
62,
34675,
796,
1364,
62,
34675,
58,
16,
25,
437,
12,
16,
60,
220,
1303,
45616,
262,
25462,
1343,
220,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
826,
9546,
532,
198,
220,
220,
220,
220,
220,
220,
220,
826,
62,
34675,
796,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
329,
4693,
62,
5420,
62,
12985,
287,
1351,
62,
1659,
62,
29498,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
4693,
4686,
290,
3995,
16590,
15748,
532,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4693,
796,
11688,
7,
35448,
62,
5420,
62,
12985,
553,
35448,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
336,
1073,
14822,
796,
11688,
7,
35448,
62,
5420,
62,
12985,
11,
366,
301,
78,
16590,
15748,
4943,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
826,
62,
34675,
1635,
28,
17971,
7,
301,
1073,
14822,
27493,
3,
7,
35448,
47762,
1,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
826,
62,
34675,
796,
826,
62,
34675,
58,
16,
25,
437,
12,
16,
60,
220,
220,
220,
1303,
2005,
1462,
487,
262,
25462,
1343,
220,
628,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
48040,
262,
6317,
2134,
532,
198,
220,
220,
220,
220,
220,
220,
220,
3857,
752,
13,
260,
2673,
62,
3672,
796,
374,
3672,
198,
220,
220,
220,
220,
220,
220,
220,
3857,
752,
13,
721,
62,
17618,
796,
12878,
30866,
198,
220,
220,
220,
220,
220,
220,
220,
3857,
752,
13,
260,
37393,
796,
2710,
62,
32109,
198,
220,
220,
220,
220,
220,
220,
220,
3857,
752,
13,
9464,
62,
34675,
796,
1364,
62,
34675,
198,
220,
220,
220,
220,
220,
220,
220,
3857,
752,
13,
3506,
62,
34675,
796,
826,
62,
34675,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
12940,
532,
198,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
260,
2673,
62,
15252,
62,
18747,
11,
3857,
752,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
1441,
532,
198,
220,
220,
220,
1441,
6317,
62,
15252,
62,
18747,
198,
437,
198,
198,
8818,
1382,
62,
14116,
75,
62,
23065,
62,
42895,
7,
21048,
62,
15763,
3712,
37643,
2538,
1732,
11,
29472,
3712,
10100,
2599,
25,
47468,
15167,
21950,
628,
220,
220,
220,
1303,
41216,
532,
198,
220,
220,
220,
1430,
62,
42895,
796,
569,
43,
15167,
21950,
3419,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
3440,
262,
13639,
2420,
329,
15326,
14990,
532,
198,
220,
220,
220,
3108,
62,
1462,
62,
23928,
796,
17971,
7,
6978,
62,
1462,
62,
26495,
20679,
17080,
3890,
14,
73,
43640,
14,
17256,
14,
29551,
14990,
12,
39681,
12,
16375,
13,
14116,
1,
198,
220,
220,
220,
11876,
796,
2291,
62,
8818,
7,
6978,
62,
1462,
62,
23928,
8,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
9807,
532,
198,
220,
220,
220,
6228,
62,
22252,
796,
13538,
198,
220,
220,
220,
685,
38568,
62,
22252,
1635,
28,
1627,
329,
1627,
287,
11876,
60,
628,
220,
220,
220,
1303,
751,
1366,
284,
1430,
62,
42895,
532,
198,
220,
220,
220,
1430,
62,
42895,
13,
34345,
796,
29472,
26,
198,
220,
220,
220,
1430,
62,
42895,
13,
22252,
796,
6228,
62,
22252,
198,
220,
220,
220,
1430,
62,
42895,
13,
4906,
796,
1058,
22252,
628,
220,
220,
220,
1303,
1441,
532,
198,
220,
220,
220,
1441,
1430,
62,
42895,
198,
437,
198,
198,
8818,
1382,
62,
2164,
77,
62,
23065,
62,
42895,
7,
21048,
62,
15763,
3712,
37643,
2538,
1732,
11,
29472,
3712,
10100,
2599,
25,
47468,
15167,
21950,
628,
220,
220,
220,
1303,
41216,
532,
198,
220,
220,
220,
1430,
62,
42895,
796,
569,
43,
15167,
21950,
3419,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
3440,
262,
13639,
2420,
329,
15326,
14990,
532,
198,
220,
220,
220,
3108,
62,
1462,
62,
23928,
796,
17971,
7,
6978,
62,
1462,
62,
26495,
20679,
17080,
3890,
14,
73,
43640,
14,
17256,
14,
10761,
45,
12,
39681,
12,
16375,
13,
14116,
1,
198,
220,
220,
220,
11876,
796,
2291,
62,
8818,
7,
6978,
62,
1462,
62,
23928,
8,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
9807,
532,
198,
220,
220,
220,
6228,
62,
22252,
796,
13538,
198,
220,
220,
220,
685,
38568,
62,
22252,
1635,
28,
1627,
329,
1627,
287,
11876,
60,
628,
220,
220,
220,
1303,
751,
1366,
284,
1430,
62,
42895,
532,
198,
220,
220,
220,
1430,
62,
42895,
13,
34345,
796,
29472,
26,
198,
220,
220,
220,
1430,
62,
42895,
13,
22252,
796,
6228,
62,
22252,
198,
220,
220,
220,
1430,
62,
42895,
13,
4906,
796,
1058,
22252,
628,
220,
220,
220,
1303,
1441,
532,
198,
220,
220,
220,
1441,
1430,
62,
42895,
198,
437,
198,
198,
8818,
1382,
62,
20541,
62,
25677,
62,
23065,
62,
42895,
7,
21048,
62,
15763,
3712,
37643,
2538,
1732,
11,
29472,
3712,
10100,
2599,
25,
47468,
15167,
21950,
628,
220,
220,
220,
1303,
41216,
532,
198,
220,
220,
220,
1430,
62,
42895,
796,
569,
43,
15167,
21950,
3419,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
3440,
262,
13639,
2420,
329,
15326,
14990,
532,
198,
220,
220,
220,
3108,
62,
1462,
62,
23928,
796,
17971,
7,
6978,
62,
1462,
62,
26495,
20679,
17080,
3890,
14,
73,
43640,
14,
17256,
14,
22289,
12,
39681,
12,
16375,
13,
14116,
1,
198,
220,
220,
220,
11876,
796,
2291,
62,
8818,
7,
6978,
62,
1462,
62,
23928,
8,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
9807,
532,
198,
220,
220,
220,
6228,
62,
22252,
796,
13538,
198,
220,
220,
220,
685,
38568,
62,
22252,
1635,
28,
1627,
329,
1627,
287,
11876,
60,
628,
220,
220,
220,
1303,
751,
1366,
284,
1430,
62,
42895,
532,
198,
220,
220,
220,
1430,
62,
42895,
13,
34345,
796,
29472,
26,
198,
220,
220,
220,
1430,
62,
42895,
13,
22252,
796,
6228,
62,
22252,
198,
220,
220,
220,
1430,
62,
42895,
13,
4906,
796,
1058,
22252,
628,
220,
220,
220,
1303,
1441,
532,
198,
220,
220,
220,
1441,
1430,
62,
42895,
198,
437,
198,
198,
8818,
1382,
62,
4164,
28426,
1042,
62,
23065,
62,
42895,
7,
21048,
62,
15763,
3712,
37643,
2538,
1732,
11,
29472,
3712,
10100,
2599,
25,
47468,
15167,
21950,
628,
220,
220,
220,
1303,
41216,
532,
198,
220,
220,
220,
11876,
796,
15690,
90,
10100,
11,
16,
92,
3419,
198,
220,
220,
220,
1430,
62,
42895,
796,
569,
43,
15167,
21950,
3419,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
13639,
1321,
532,
198,
220,
220,
220,
1343,
7,
22252,
11,
366,
1003,
41906,
17174,
4557,
35625,
3373,
59,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
25113,
47123,
6242,
3535,
31125,
3712,
2257,
7227,
59,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
366,
1003,
3395,
28426,
1042,
1700,
5794,
7479,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
366,
1003,
6317,
62,
3672,
357,
34642,
828,
685,
90,
26,
46728,
863,
900,
286,
9940,
3146,
930,
17635,
92,
4357,
45018,
415,
62,
8841,
11,
11167,
62,
8841,
11,
260,
37393,
59,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
553,
1003,
59,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
366,
1003,
14252,
7479,
77,
15341,
198,
220,
220,
220,
1343,
7,
22252,
11,
366,
1003,
383,
6317,
62,
3672,
2214,
318,
3748,
11,
290,
14623,
578,
14354,
460,
407,
423,
2041,
34534,
393,
9029,
59,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
366,
1003,
59,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
366,
1003,
17934,
7479,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
366,
1003,
371,
62,
32,
62,
28869,
62,
17,
17414,
21,
13,
18,
13,
19,
13,
1485,
4357,
44,
62,
265,
79,
62,
66,
10,
44,
62,
20,
79,
17457,
430,
10,
44,
62,
10853,
62,
43,
62,
66,
11,
44,
62,
324,
79,
62,
66,
10,
44,
62,
14415,
62,
66,
10,
44,
62,
4563,
62,
66,
11,
9562,
59,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
366,
1003,
59,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
366,
1003,
520,
5374,
72,
16996,
44036,
389,
662,
12,
79,
1631,
284,
14623,
578,
6194,
11,
329,
1672,
7479,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
366,
1003,
371,
62,
24411,
36,
17414,
16,
13,
17,
13,
16,
13,
940,
26,
352,
13,
16,
13,
16,
13,
16,
4357,
44,
62,
8679,
64,
62,
66,
10,
17,
9,
44,
62,
71,
62,
66,
10,
17,
9,
44,
62,
77,
24411,
62,
66,
11,
44,
62,
1073,
64,
62,
66,
10,
44,
62,
316,
1219,
62,
66,
10,
17,
9,
44,
62,
77,
324,
62,
66,
11,
7942,
59,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
37082,
77,
4943,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
1382,
262,
6317,
7177,
286,
5563,
532,
198,
220,
220,
220,
6317,
62,
15252,
62,
18747,
796,
1382,
62,
4164,
29304,
62,
260,
2673,
62,
15252,
62,
18747,
7,
21048,
62,
15763,
8,
198,
220,
220,
220,
45218,
62,
8841,
796,
13538,
198,
220,
220,
220,
329,
6317,
62,
15252,
3712,
47468,
9171,
29304,
3041,
2673,
287,
6317,
62,
15252,
62,
18747,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
651,
1366,
532,
198,
220,
220,
220,
220,
220,
220,
220,
6317,
62,
3672,
796,
6317,
62,
15252,
13,
260,
2673,
62,
3672,
198,
220,
220,
220,
220,
220,
220,
220,
9940,
62,
17618,
796,
6317,
62,
15252,
13,
721,
62,
17618,
198,
220,
220,
220,
220,
220,
220,
220,
1364,
62,
34675,
796,
6317,
62,
15252,
13,
9464,
62,
34675,
198,
220,
220,
220,
220,
220,
220,
220,
826,
62,
34675,
796,
6317,
62,
15252,
13,
3506,
62,
34675,
198,
220,
220,
220,
220,
220,
220,
220,
48287,
62,
32109,
796,
6317,
62,
15252,
13,
260,
37393,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
1382,
257,
6317,
4731,
532,
198,
220,
220,
220,
220,
220,
220,
220,
45218,
62,
8841,
796,
17971,
7,
260,
2673,
62,
3672,
828,
3,
7,
721,
62,
17618,
828,
3,
7,
9464,
62,
34675,
828,
3,
7,
3506,
62,
34675,
828,
3,
7,
260,
37393,
62,
32109,
19415,
77,
1,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
4574,
4291,
262,
11876,
532,
198,
220,
220,
220,
220,
220,
220,
220,
1343,
7,
22252,
11,
45218,
62,
8841,
8,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
1598,
532,
198,
220,
220,
220,
220,
220,
220,
220,
45218,
62,
8841,
796,
17635,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
1969,
262,
2665,
532,
198,
220,
220,
220,
1343,
7,
22252,
11,
37082,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
25113,
47123,
6242,
3535,
31125,
3712,
2257,
3185,
59,
77,
4943,
198,
220,
220,
220,
1343,
7,
22252,
11,
366,
1003,
41906,
17174,
4557,
35625,
3373,
59,
77,
4943,
628,
220,
220,
220,
1303,
9807,
532,
198,
220,
220,
220,
6228,
62,
22252,
796,
13538,
198,
220,
220,
220,
685,
38568,
62,
22252,
1635,
28,
1627,
329,
1627,
287,
11876,
60,
628,
220,
220,
220,
1303,
751,
1366,
284,
1430,
62,
42895,
532,
198,
220,
220,
220,
1430,
62,
42895,
13,
34345,
796,
29472,
26,
198,
220,
220,
220,
1430,
62,
42895,
13,
22252,
796,
6228,
62,
22252,
198,
220,
220,
220,
1430,
62,
42895,
13,
4906,
796,
1058,
22252,
628,
220,
220,
220,
1303,
1441,
532,
198,
220,
220,
220,
1441,
1430,
62,
42895,
198,
437
] | 2.558325 | 3,009 |
const allpermorder = [:NATURAL, :ENTROPIC, :REV_ENTROPIC, :RANDOM]
"""
ardca(Z::Array{Ti,2},W::Vector{Float64}; kwds...)
Auto-regressive analysis on the L×M alignment `Z` (numerically encoded in 1,…,21), and the `M`-dimensional normalized
weight vector `W`.
Return two `struct`: `::ArNet` (containing the inferred hyperparameters) and `::ArVar`
Optional arguments:
* `lambdaJ::Real=0.01` coupling L₂ regularization parameter (lagrange multiplier)
* `lambdaH::Real=0.01` field L₂ regularization parameter (lagrange multiplier)
* `epsconv::Real=1.0e-5` convergence value in minimzation
* `maxit::Int=1000` maximum number of iteration in minimization
* `verbose::Bool=true` set to `false` to stop printing convergence info on `stdout`
* `method::Symbol=:LD_LBFGS` optimization strategy see [`NLopt.jl`](https://github.com/JuliaOpt/NLopt.jl) for other options
* `permorder::Union{Symbol,Vector{Ti}}=:ENTROPIC` permutation order. Possible values are `:NATURAL,:ENTROPIC,:REV_ENTROPIC,:RANDOM` or a custom permutation vector
# Examples
```
julia> arnet, arvar= ardca(Z,W,lambdaJ=0,lambdaH=0,permorder=:REV_ENTROPIC,epsconv=1e-12);
```
"""
function ardca(Z::Array{Ti,2},W::Vector{Float64};
lambdaJ::Real=0.01,
lambdaH::Real=0.01,
epsconv::Real=1.0e-5,
maxit::Int=1000,
verbose::Bool=true,
method::Symbol=:LD_LBFGS,
permorder::Union{Symbol,Vector{Int}}=:ENTROPIC
) where Ti <: Integer
checkpermorder(permorder)
all(x -> x > 0, W) || throw(DomainError("vector W should normalized and with all positive elements"))
isapprox(sum(W), 1) || throw(DomainError("sum(W) ≠ 1. Consider normalizing the vector W"))
N, M = size(Z)
M = length(W)
q = Int(maximum(Z))
aralg = ArAlg(method, verbose, epsconv, maxit)
arvar = ArVar(N, M, q, lambdaJ, lambdaH, Z, W, permorder)
θ,psval = minimize_arnet(aralg, arvar)
Base.GC.gc() # something wrong with SharedArrays on Mac
ArNet(θ,arvar),arvar
end
"""
ardca(filename::String; kwds...)
Run [`ardca`](@ref) on the fasta alignment in `filename`
Return two `struct`: `::ArNet` (containing the inferred hyperparameters) and `::ArVar`
Optional arguments:
* `max_gap_fraction::Real=0.9` maximum fraction of insert in the sequence
* `remove_dups::Bool=true` if `true` remove duplicated sequences
* `theta=:auto` if `:auto` compute reweighint automatically. Otherwise set a `Float64` value `0 ≤ theta ≤ 1`
* `lambdaJ::Real=0.01` coupling L₂ regularization parameter (lagrange multiplier)
* `lambdaH::Real=0.01` field L₂ regularization parameter (lagrange multiplier)
* `epsconv::Real=1.0e-5` convergence value in minimzation
* `maxit::Int=1000` maximum number of iteration in minimization
* `verbose::Bool=true` set to `false` to stop printing convergence info on `stdout`
* `method::Symbol=:LD_LBFGS` optimization strategy see [`NLopt.jl`](https://github.com/JuliaOpt/NLopt.jl) for other options
* `permorder::Union{Symbol,Vector{Ti}}=:ENTROPIC` permutation order. Possible
values are `:NATURAL,:ENTROPIC,:REV_ENTROPIC,:RANDOM` or a custom permutation
vector
# Examples
```
julia> arnet, arvar = ardca("pf14.fasta", permorder=:ENTROPIC)
```
"""
function ardca(filename::String;
theta::Union{Symbol,Real}=:auto,
max_gap_fraction::Real=0.9,
remove_dups::Bool=true,
kwds...)
W, Z, N, M, q = read_fasta(filename, max_gap_fraction, theta, remove_dups)
W ./= sum(W)
ardca(Z, W; kwds...)
end
function checkpermorder(po::Symbol)
po ∈ allpermorder || error("permorder :$po not iplemented: only $allpermorder are defined");
end
(checkpermorder(po::Vector{Ti}) where Ti <: Integer) = isperm(po) || error("permorder is not a permutation")
function minimize_arnet(alg::ArAlg, var::ArVar{Ti}) where Ti
@extract var : N q q2
@extract alg : epsconv maxit method
vecps = Vector{Float64}(undef,N - 1)
θ = Vector{Float64}(undef, ((N*(N-1))>>1)*q2 + (N-1)*q)
Threads.@threads for site in 1:N-1
x0 = zeros(Float64, site * q2 + q)
opt = Opt(method, length(x0))
ftol_abs!(opt, epsconv)
xtol_rel!(opt, epsconv)
xtol_abs!(opt, epsconv)
ftol_rel!(opt, epsconv)
maxeval!( opt, maxit)
min_objective!(opt, (x, g) -> optimfunwrapper(x, g, site, var))
elapstime = @elapsed (minf, minx, ret) = optimize(opt, x0)
alg.verbose && @printf("site = %d\tpl = %.4f\ttime = %.4f\t", site, minf, elapstime)
alg.verbose && println("status = $ret")
vecps[site] = minf
offset = div(site*(site-1),2)*q2 + (site-1)*q + 1
θ[offset:offset+site * q2 + q - 1] .= minx
end
return θ, vecps
end
function optimfunwrapper(x::Vector, g::Vector, site, var)
g === nothing && (g = zeros(Float64, length(x)))
return pslikeandgrad!(x, g, site, var)
end
function pslikeandgrad!(x::Vector{Float64}, grad::Vector{Float64}, site::Int, arvar::ArVar)
@extract arvar : N M q q2 lambdaJ lambdaH Z W IdxZ
LL = length(x)
for i = 1:LL - q
grad[i] = 2.0 * lambdaJ * x[i]
end
for i = (LL - q + 1):LL
grad[i] = 2.0 * lambdaH * x[i]
end
pseudolike = 0.0
vecene = zeros(Float64, q)
expvecenesumnorm = zeros(Float64, q)
@inbounds for m in 1:M
izm = view(IdxZ, :, m)
zsm = Z[site+1,m] # the i index of P(x_i|x_1,...,x_i-1) corresponds here to i+1
fillvecene!(vecene, x, site, izm, q, N)
lnorm = logsumexp(vecene)
expvecenesumnorm .= @. exp(vecene - lnorm)
pseudolike -= W[m] * (vecene[ zsm ] - lnorm)
sq2 = site * q2
@avx for i in 1:site
for s in 1:q
grad[ izm[i] + s ] += W[m] * expvecenesumnorm[s]
end
grad[ izm[i] + zsm ] -= W[m]
end
@avx for s = 1:q
grad[ sq2 + s ] += W[m] * expvecenesumnorm[s]
end
grad[ sq2 + zsm ] -= W[m]
end
pseudolike += l2norm_asym(x, arvar)
end
function fillvecene!(vecene::Vector{Float64}, x::Vector{Float64}, site::Int, IdxSeq::AbstractArray{Int,1}, q::Int, N::Int)
q2 = q^2
sq2 = site * q2
@inbounds for l in 1:q
scra = 0.0
@avx for i in 1:site
scra += x[IdxSeq[i] + l]
end
scra += x[sq2 + l] # sum H
vecene[l] = scra
end
end
function logsumexp(X::Vector)
u = maximum(X)
isfinite(u) || return float(u)
return u + log(sum(x -> exp(x - u), X))
end
function l2norm_asym(vec::Array{Float64,1}, arvar::ArVar)
@extract arvar : q N lambdaJ lambdaH
LL = length(vec)
mysum1 = 0.0
@inbounds @avx for i = 1:(LL - q)
mysum1 += vec[i] * vec[i]
end
mysum1 *= lambdaJ
mysum2 = 0.0
@inbounds @avx for i = (LL - q + 1):LL
mysum2 += vec[i] * vec[i]
end
mysum2 *= lambdaH
return mysum1 + mysum2
end | [
9979,
477,
16321,
2875,
796,
685,
25,
34259,
4261,
1847,
11,
1058,
3525,
49,
3185,
2149,
11,
1058,
2200,
53,
62,
3525,
49,
3185,
2149,
11,
1058,
49,
6981,
2662,
60,
198,
37811,
198,
220,
220,
220,
33848,
6888,
7,
57,
3712,
19182,
90,
40533,
11,
17,
5512,
54,
3712,
38469,
90,
43879,
2414,
19629,
479,
86,
9310,
23029,
198,
27722,
12,
2301,
3314,
3781,
319,
262,
406,
12906,
44,
19114,
4600,
57,
63,
357,
77,
6975,
1146,
30240,
287,
352,
11,
1399,
11,
2481,
828,
290,
262,
4600,
44,
63,
12,
19577,
39279,
220,
198,
6551,
15879,
4600,
54,
44646,
198,
198,
13615,
734,
4600,
7249,
63,
25,
4600,
3712,
3163,
7934,
63,
357,
38301,
262,
41240,
8718,
17143,
7307,
8,
290,
4600,
3712,
3163,
19852,
63,
198,
198,
30719,
7159,
25,
198,
9,
4600,
50033,
41,
3712,
15633,
28,
15,
13,
486,
63,
40204,
406,
158,
224,
224,
3218,
1634,
11507,
357,
30909,
9521,
33090,
8,
198,
9,
4600,
50033,
39,
3712,
15633,
28,
15,
13,
486,
63,
2214,
406,
158,
224,
224,
3218,
1634,
11507,
357,
30909,
9521,
33090,
8,
198,
9,
4600,
25386,
42946,
3712,
15633,
28,
16,
13,
15,
68,
12,
20,
63,
40826,
1988,
287,
10356,
89,
341,
198,
9,
4600,
9806,
270,
3712,
5317,
28,
12825,
63,
5415,
1271,
286,
24415,
287,
10356,
1634,
198,
9,
4600,
19011,
577,
3712,
33,
970,
28,
7942,
63,
900,
284,
4600,
9562,
63,
284,
2245,
13570,
40826,
7508,
319,
4600,
19282,
448,
63,
198,
9,
4600,
24396,
3712,
13940,
23650,
28,
25,
11163,
62,
43,
29499,
14313,
63,
23989,
4811,
766,
685,
63,
32572,
8738,
13,
20362,
63,
16151,
5450,
1378,
12567,
13,
785,
14,
16980,
544,
27871,
14,
32572,
8738,
13,
20362,
8,
329,
584,
3689,
198,
9,
4600,
16321,
2875,
3712,
38176,
90,
13940,
23650,
11,
38469,
90,
40533,
11709,
28,
25,
3525,
49,
3185,
2149,
63,
9943,
7094,
1502,
13,
33671,
3815,
389,
4600,
25,
34259,
4261,
1847,
11,
25,
3525,
49,
3185,
2149,
11,
25,
2200,
53,
62,
3525,
49,
3185,
2149,
11,
25,
49,
6981,
2662,
63,
393,
257,
2183,
9943,
7094,
15879,
198,
198,
2,
21066,
198,
15506,
63,
198,
73,
43640,
29,
610,
3262,
11,
610,
7785,
28,
33848,
6888,
7,
57,
11,
54,
11,
50033,
41,
28,
15,
11,
50033,
39,
28,
15,
11,
16321,
2875,
28,
25,
2200,
53,
62,
3525,
49,
3185,
2149,
11,
25386,
42946,
28,
16,
68,
12,
1065,
1776,
198,
15506,
63,
198,
37811,
198,
8818,
33848,
6888,
7,
57,
3712,
19182,
90,
40533,
11,
17,
5512,
54,
3712,
38469,
90,
43879,
2414,
19629,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
37456,
41,
3712,
15633,
28,
15,
13,
486,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
37456,
39,
3712,
15633,
28,
15,
13,
486,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
304,
862,
42946,
3712,
15633,
28,
16,
13,
15,
68,
12,
20,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3509,
270,
3712,
5317,
28,
12825,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
15942,
577,
3712,
33,
970,
28,
7942,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2446,
3712,
13940,
23650,
28,
25,
11163,
62,
43,
29499,
14313,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
9943,
2875,
3712,
38176,
90,
13940,
23650,
11,
38469,
90,
5317,
11709,
28,
25,
3525,
49,
3185,
2149,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1267,
810,
16953,
1279,
25,
34142,
628,
220,
220,
220,
2198,
16321,
2875,
7,
16321,
2875,
8,
198,
220,
220,
220,
477,
7,
87,
4613,
2124,
1875,
657,
11,
370,
8,
8614,
3714,
7,
43961,
12331,
7203,
31364,
370,
815,
39279,
290,
351,
477,
3967,
4847,
48774,
198,
220,
220,
220,
318,
1324,
13907,
7,
16345,
7,
54,
828,
352,
8,
8614,
3714,
7,
43961,
12331,
7203,
16345,
7,
54,
8,
15139,
254,
352,
13,
12642,
3487,
2890,
262,
15879,
370,
48774,
198,
220,
220,
220,
399,
11,
337,
796,
2546,
7,
57,
8,
198,
220,
220,
220,
337,
796,
4129,
7,
54,
8,
198,
220,
220,
220,
10662,
796,
2558,
7,
47033,
7,
57,
4008,
198,
220,
220,
220,
610,
14016,
796,
943,
2348,
70,
7,
24396,
11,
15942,
577,
11,
304,
862,
42946,
11,
3509,
270,
8,
198,
220,
220,
220,
610,
7785,
796,
943,
19852,
7,
45,
11,
337,
11,
10662,
11,
37456,
41,
11,
37456,
39,
11,
1168,
11,
370,
11,
9943,
2875,
8,
198,
220,
220,
220,
7377,
116,
11,
862,
2100,
796,
17775,
62,
1501,
316,
7,
283,
14016,
11,
610,
7785,
8,
198,
220,
220,
220,
7308,
13,
15916,
13,
36484,
3419,
1303,
1223,
2642,
351,
39403,
3163,
20477,
319,
4100,
198,
220,
220,
220,
943,
7934,
7,
138,
116,
11,
283,
7785,
828,
283,
7785,
198,
437,
198,
37811,
198,
220,
220,
220,
33848,
6888,
7,
34345,
3712,
10100,
26,
479,
86,
9310,
23029,
198,
10987,
685,
63,
446,
6888,
63,
16151,
31,
5420,
8,
319,
262,
3049,
64,
19114,
287,
4600,
34345,
63,
198,
198,
13615,
734,
4600,
7249,
63,
25,
4600,
3712,
3163,
7934,
63,
357,
38301,
262,
41240,
8718,
17143,
7307,
8,
290,
4600,
3712,
3163,
19852,
63,
198,
198,
30719,
7159,
25,
198,
9,
4600,
9806,
62,
43554,
62,
69,
7861,
3712,
15633,
28,
15,
13,
24,
63,
5415,
13390,
286,
7550,
287,
262,
8379,
198,
9,
4600,
28956,
62,
646,
862,
3712,
33,
970,
28,
7942,
63,
611,
4600,
7942,
63,
4781,
14184,
3474,
16311,
198,
9,
4600,
1169,
8326,
28,
25,
23736,
63,
611,
4600,
25,
23736,
63,
24061,
302,
732,
394,
600,
6338,
13,
15323,
900,
257,
4600,
43879,
2414,
63,
1988,
4600,
15,
41305,
262,
8326,
41305,
352,
63,
198,
9,
4600,
50033,
41,
3712,
15633,
28,
15,
13,
486,
63,
40204,
406,
158,
224,
224,
3218,
1634,
11507,
357,
30909,
9521,
33090,
8,
198,
9,
4600,
50033,
39,
3712,
15633,
28,
15,
13,
486,
63,
2214,
406,
158,
224,
224,
3218,
1634,
11507,
357,
30909,
9521,
33090,
8,
198,
9,
4600,
25386,
42946,
3712,
15633,
28,
16,
13,
15,
68,
12,
20,
63,
40826,
1988,
287,
10356,
89,
341,
198,
9,
4600,
9806,
270,
3712,
5317,
28,
12825,
63,
5415,
1271,
286,
24415,
287,
10356,
1634,
198,
9,
4600,
19011,
577,
3712,
33,
970,
28,
7942,
63,
900,
284,
4600,
9562,
63,
284,
2245,
13570,
40826,
7508,
319,
4600,
19282,
448,
63,
198,
9,
4600,
24396,
3712,
13940,
23650,
28,
25,
11163,
62,
43,
29499,
14313,
63,
23989,
4811,
766,
685,
63,
32572,
8738,
13,
20362,
63,
16151,
5450,
1378,
12567,
13,
785,
14,
16980,
544,
27871,
14,
32572,
8738,
13,
20362,
8,
329,
584,
3689,
198,
9,
4600,
16321,
2875,
3712,
38176,
90,
13940,
23650,
11,
38469,
90,
40533,
11709,
28,
25,
3525,
49,
3185,
2149,
63,
9943,
7094,
1502,
13,
33671,
198,
220,
3815,
389,
4600,
25,
34259,
4261,
1847,
11,
25,
3525,
49,
3185,
2149,
11,
25,
2200,
53,
62,
3525,
49,
3185,
2149,
11,
25,
49,
6981,
2662,
63,
393,
257,
2183,
9943,
7094,
198,
220,
15879,
198,
198,
2,
21066,
198,
15506,
63,
198,
73,
43640,
29,
610,
3262,
11,
610,
7785,
796,
220,
33848,
6888,
7203,
79,
69,
1415,
13,
7217,
64,
1600,
9943,
2875,
28,
25,
3525,
49,
3185,
2149,
8,
198,
15506,
63,
198,
37811,
198,
8818,
33848,
6888,
7,
34345,
3712,
10100,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
262,
8326,
3712,
38176,
90,
13940,
23650,
11,
15633,
92,
28,
25,
23736,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3509,
62,
43554,
62,
69,
7861,
3712,
15633,
28,
15,
13,
24,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4781,
62,
646,
862,
3712,
33,
970,
28,
7942,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
479,
86,
9310,
23029,
198,
220,
220,
220,
370,
11,
1168,
11,
399,
11,
337,
11,
10662,
796,
1100,
62,
7217,
64,
7,
34345,
11,
3509,
62,
43554,
62,
69,
7861,
11,
262,
8326,
11,
4781,
62,
646,
862,
8,
198,
220,
220,
220,
370,
24457,
28,
2160,
7,
54,
8,
198,
220,
220,
220,
33848,
6888,
7,
57,
11,
370,
26,
479,
86,
9310,
23029,
198,
437,
198,
198,
8818,
2198,
16321,
2875,
7,
7501,
3712,
13940,
23650,
8,
198,
220,
220,
220,
745,
18872,
230,
477,
16321,
2875,
8614,
4049,
7203,
16321,
2875,
1058,
3,
7501,
407,
20966,
1732,
276,
25,
691,
720,
439,
16321,
2875,
389,
5447,
15341,
198,
437,
198,
198,
7,
9122,
16321,
2875,
7,
7501,
3712,
38469,
90,
40533,
30072,
810,
16953,
1279,
25,
34142,
8,
796,
318,
16321,
7,
7501,
8,
8614,
4049,
7203,
16321,
2875,
318,
407,
257,
9943,
7094,
4943,
198,
198,
8818,
17775,
62,
1501,
316,
7,
14016,
3712,
3163,
2348,
70,
11,
1401,
3712,
3163,
19852,
90,
40533,
30072,
810,
16953,
198,
220,
220,
220,
2488,
2302,
974,
1401,
1058,
399,
10662,
10662,
17,
198,
220,
220,
220,
2488,
2302,
974,
435,
70,
1058,
304,
862,
42946,
3509,
270,
2446,
198,
220,
220,
220,
43030,
862,
796,
20650,
90,
43879,
2414,
92,
7,
917,
891,
11,
45,
532,
352,
8,
198,
220,
220,
220,
7377,
116,
796,
20650,
90,
43879,
2414,
92,
7,
917,
891,
11,
14808,
45,
9,
7,
45,
12,
16,
4008,
4211,
16,
27493,
80,
17,
1343,
357,
45,
12,
16,
27493,
80,
8,
198,
220,
220,
220,
14122,
82,
13,
31,
16663,
82,
329,
2524,
287,
352,
25,
45,
12,
16,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
15,
796,
1976,
27498,
7,
43879,
2414,
11,
2524,
1635,
10662,
17,
1343,
10662,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2172,
796,
13123,
7,
24396,
11,
4129,
7,
87,
15,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
10117,
349,
62,
8937,
0,
7,
8738,
11,
304,
862,
42946,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
742,
349,
62,
2411,
0,
7,
8738,
11,
304,
862,
42946,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
742,
349,
62,
8937,
0,
7,
8738,
11,
304,
862,
42946,
8,
198,
220,
220,
220,
220,
220,
220,
220,
10117,
349,
62,
2411,
0,
7,
8738,
11,
304,
862,
42946,
8,
198,
220,
220,
220,
220,
220,
220,
220,
3509,
18206,
0,
7,
2172,
11,
3509,
270,
8,
198,
220,
220,
220,
220,
220,
220,
220,
949,
62,
15252,
425,
0,
7,
8738,
11,
357,
87,
11,
308,
8,
4613,
6436,
12543,
48553,
7,
87,
11,
308,
11,
2524,
11,
1401,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
1288,
499,
301,
524,
796,
2488,
417,
28361,
220,
357,
1084,
69,
11,
949,
87,
11,
1005,
8,
796,
27183,
7,
8738,
11,
2124,
15,
8,
198,
220,
220,
220,
220,
220,
220,
220,
435,
70,
13,
19011,
577,
11405,
2488,
37435,
7203,
15654,
796,
4064,
67,
59,
83,
489,
796,
4064,
13,
19,
69,
59,
926,
524,
796,
4064,
13,
19,
69,
59,
83,
1600,
2524,
11,
949,
69,
11,
1288,
499,
301,
524,
8,
198,
220,
220,
220,
220,
220,
220,
220,
435,
70,
13,
19011,
577,
11405,
44872,
7203,
13376,
796,
720,
1186,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
43030,
862,
58,
15654,
60,
796,
949,
69,
198,
220,
220,
220,
220,
220,
220,
220,
11677,
796,
2659,
7,
15654,
9,
7,
15654,
12,
16,
828,
17,
27493,
80,
17,
1343,
357,
15654,
12,
16,
27493,
80,
1343,
352,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
116,
58,
28968,
25,
28968,
10,
15654,
1635,
10662,
17,
1343,
10662,
532,
352,
60,
764,
28,
949,
87,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
7377,
116,
11,
43030,
862,
198,
437,
198,
198,
8818,
6436,
12543,
48553,
7,
87,
3712,
38469,
11,
308,
3712,
38469,
11,
2524,
11,
1401,
8,
198,
220,
220,
220,
308,
24844,
2147,
11405,
357,
70,
796,
1976,
27498,
7,
43879,
2414,
11,
4129,
7,
87,
22305,
198,
220,
220,
220,
1441,
26692,
2339,
392,
9744,
0,
7,
87,
11,
308,
11,
2524,
11,
220,
1401,
8,
198,
437,
198,
198,
8818,
26692,
2339,
392,
9744,
0,
7,
87,
3712,
38469,
90,
43879,
2414,
5512,
3915,
3712,
38469,
90,
43879,
2414,
5512,
2524,
3712,
5317,
11,
610,
7785,
3712,
3163,
19852,
8,
198,
220,
220,
220,
2488,
2302,
974,
610,
7785,
1058,
399,
337,
10662,
10662,
17,
37456,
41,
37456,
39,
1168,
370,
5121,
87,
57,
198,
220,
220,
220,
27140,
796,
4129,
7,
87,
8,
198,
220,
220,
220,
329,
1312,
796,
352,
25,
3069,
532,
10662,
198,
220,
220,
220,
220,
220,
220,
220,
3915,
58,
72,
60,
796,
362,
13,
15,
1635,
37456,
41,
220,
1635,
2124,
58,
72,
60,
198,
220,
220,
220,
886,
198,
220,
220,
220,
329,
1312,
796,
357,
3069,
532,
10662,
1343,
352,
2599,
3069,
198,
220,
220,
220,
220,
220,
220,
220,
3915,
58,
72,
60,
796,
362,
13,
15,
1635,
37456,
39,
1635,
2124,
58,
72,
60,
198,
220,
220,
220,
886,
198,
220,
220,
220,
25038,
349,
522,
796,
657,
13,
15,
198,
220,
220,
220,
43030,
1734,
796,
1976,
27498,
7,
43879,
2414,
11,
10662,
8,
198,
220,
220,
220,
1033,
35138,
18719,
4182,
579,
796,
1976,
27498,
7,
43879,
2414,
11,
10662,
8,
198,
220,
220,
220,
2488,
259,
65,
3733,
329,
285,
287,
352,
25,
44,
198,
220,
220,
220,
220,
220,
220,
220,
220,
528,
76,
796,
1570,
7,
7390,
87,
57,
11,
1058,
11,
285,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1976,
5796,
796,
1168,
58,
15654,
10,
16,
11,
76,
60,
1303,
262,
1312,
6376,
286,
350,
7,
87,
62,
72,
91,
87,
62,
16,
42303,
11,
87,
62,
72,
12,
16,
8,
24866,
994,
284,
1312,
10,
16,
198,
220,
220,
220,
220,
220,
220,
220,
6070,
35138,
1734,
0,
7,
35138,
1734,
11,
2124,
11,
2524,
11,
220,
528,
76,
11,
10662,
11,
399,
8,
198,
220,
220,
220,
220,
220,
220,
220,
300,
27237,
796,
2604,
16345,
11201,
7,
35138,
1734,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1033,
35138,
18719,
4182,
579,
764,
28,
2488,
13,
1033,
7,
35138,
1734,
532,
300,
27237,
8,
198,
220,
220,
220,
220,
220,
220,
220,
25038,
349,
522,
48185,
370,
58,
76,
60,
1635,
357,
35138,
1734,
58,
1976,
5796,
2361,
532,
300,
27237,
8,
198,
220,
220,
220,
220,
220,
220,
220,
19862,
17,
796,
2524,
1635,
10662,
17,
220,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
615,
87,
329,
1312,
287,
352,
25,
15654,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
329,
264,
287,
352,
25,
80,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3915,
58,
220,
528,
76,
58,
72,
60,
1343,
264,
2361,
15853,
370,
58,
76,
60,
1635,
1033,
35138,
18719,
4182,
579,
58,
82,
60,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3915,
58,
220,
528,
76,
58,
72,
60,
1343,
1976,
5796,
2361,
48185,
370,
58,
76,
60,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
615,
87,
329,
264,
796,
352,
25,
80,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3915,
58,
19862,
17,
1343,
264,
2361,
15853,
370,
58,
76,
60,
1635,
1033,
35138,
18719,
4182,
579,
58,
82,
60,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
3915,
58,
19862,
17,
1343,
1976,
5796,
2361,
48185,
370,
58,
76,
60,
198,
220,
220,
220,
886,
198,
220,
220,
220,
25038,
349,
522,
15853,
300,
17,
27237,
62,
4107,
76,
7,
87,
11,
610,
7785,
8,
198,
437,
198,
198,
8818,
6070,
35138,
1734,
0,
7,
35138,
1734,
3712,
38469,
90,
43879,
2414,
5512,
2124,
3712,
38469,
90,
43879,
2414,
5512,
2524,
3712,
5317,
11,
5121,
87,
4653,
80,
3712,
23839,
19182,
90,
5317,
11,
16,
5512,
10662,
3712,
5317,
11,
399,
3712,
5317,
8,
198,
220,
220,
220,
10662,
17,
796,
10662,
61,
17,
198,
220,
220,
220,
19862,
17,
796,
2524,
1635,
10662,
17,
220,
198,
220,
220,
220,
2488,
259,
65,
3733,
329,
300,
287,
352,
25,
80,
198,
220,
220,
220,
220,
220,
220,
220,
19320,
796,
657,
13,
15,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
615,
87,
329,
1312,
287,
352,
25,
15654,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
19320,
15853,
2124,
58,
7390,
87,
4653,
80,
58,
72,
60,
1343,
300,
60,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
19320,
15853,
2124,
58,
31166,
17,
1343,
300,
60,
1303,
2160,
367,
198,
220,
220,
220,
220,
220,
220,
220,
43030,
1734,
58,
75,
60,
796,
19320,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
2604,
16345,
11201,
7,
55,
3712,
38469,
8,
198,
220,
220,
220,
334,
796,
5415,
7,
55,
8,
198,
220,
220,
220,
318,
69,
9504,
7,
84,
8,
8614,
1441,
12178,
7,
84,
8,
198,
220,
220,
220,
1441,
334,
1343,
2604,
7,
16345,
7,
87,
4613,
1033,
7,
87,
532,
334,
828,
1395,
4008,
198,
437,
198,
198,
8818,
300,
17,
27237,
62,
4107,
76,
7,
35138,
3712,
19182,
90,
43879,
2414,
11,
16,
5512,
610,
7785,
3712,
3163,
19852,
8,
198,
220,
220,
220,
2488,
2302,
974,
610,
7785,
1058,
10662,
399,
37456,
41,
37456,
39,
198,
220,
220,
220,
27140,
796,
4129,
7,
35138,
8,
198,
220,
220,
220,
616,
16345,
16,
796,
657,
13,
15,
198,
220,
220,
220,
2488,
259,
65,
3733,
2488,
615,
87,
329,
1312,
796,
352,
37498,
3069,
532,
10662,
8,
198,
220,
220,
220,
220,
220,
220,
220,
616,
16345,
16,
15853,
43030,
58,
72,
60,
1635,
43030,
58,
72,
60,
198,
220,
220,
220,
886,
198,
220,
220,
220,
616,
16345,
16,
1635,
28,
37456,
41,
198,
220,
220,
220,
616,
16345,
17,
796,
657,
13,
15,
198,
220,
220,
220,
2488,
259,
65,
3733,
2488,
615,
87,
329,
1312,
796,
357,
3069,
532,
10662,
1343,
352,
2599,
3069,
198,
220,
220,
220,
220,
220,
220,
220,
616,
16345,
17,
15853,
43030,
58,
72,
60,
1635,
43030,
58,
72,
60,
198,
220,
220,
220,
886,
198,
220,
220,
220,
616,
16345,
17,
1635,
28,
37456,
39,
198,
220,
220,
220,
1441,
616,
16345,
16,
1343,
616,
16345,
17,
198,
437
] | 2.173683 | 3,207 |
using ApproxFun, LinearAlgebra, Plots
# In this example, we solve the heat equation with left Neumann and
# right Dirichlet boundary conditions.
# To allow for a solution through matrix exponentiation, we approximate
# the initial condition with a basis that automatically constructs
# even and odd extensions on the left and right boundaries respectively. (Method of images)
n = 100
xlim = 10
xarray = (chebyshevpoints(n) .+ 1) .* xlim/2 # Chebyshev points on 0..xlim
r = 1.0 # right boundary value (left is assumed to be zero)
S = CosSpace(0..4*xlim)
u₀(x) = atan(x)*(π + atan(x-9) + atan(5-x))/π^2 - r # subtract by `r` to homogenise the right BC
plot(xarray, u₀)
v = u₀.(xarray) # values to use in fitting
m = div(n,2) # m << n for regularisation
# Create a Vandermonde matrix by evaluating the basis at the grid:
# We ensure the basis satisfies the boundary conditions by using
# the entries corresponding to {cos(x), cos(3x), cos(5x), ...}.
V = zeros(n, m)
for k = 1:m
V[:,k] = Fun(S,[zeros(2*k-1);1]).(xarray)
end
c₀ = zeros(2*m)
c₀[2:2:end] = V\v # do the fit
f = Fun(S, c₀) # Likely not a good approximation due to boundary discontinuities, but high frequencies will disappear quickly anyway
plot([xarray.+10; xarray; xarray.-10], f, xlims=(-5, 15))
scatter!(xarray, u₀, markersize=0.2)
L = Derivative(S, 2)
L = Matrix(L[1:2m,1:2m])
@manipulate for T=[zeros(5); 0.0:0.01:1]
u_t = Fun(S, exp(T*L)*c₀) + r # calculate solution and add back homogenisation term
plot(-0:0.05:10, u_t, ylims=(0,1), legend=:none)
end
@gif for T=[zeros(5); 0.0:0.5:50]
u_t = Fun(S, exp(T*L)*c₀) + r # calculate solution and add back homogenisation term
plot(-0:0.05:10, u_t, ylims=(0,1), legend=:none)
end
| [
3500,
2034,
13907,
24629,
11,
44800,
2348,
29230,
11,
1345,
1747,
198,
198,
2,
554,
428,
1672,
11,
356,
8494,
262,
4894,
16022,
351,
1364,
3169,
40062,
290,
198,
2,
826,
36202,
488,
1616,
18645,
3403,
13,
198,
2,
1675,
1249,
329,
257,
4610,
832,
17593,
28622,
3920,
11,
356,
27665,
198,
2,
262,
4238,
4006,
351,
257,
4308,
326,
6338,
34175,
198,
2,
772,
290,
5629,
18366,
319,
262,
1364,
290,
826,
13215,
8148,
13,
357,
17410,
286,
4263,
8,
198,
198,
77,
796,
1802,
198,
87,
2475,
796,
838,
198,
87,
18747,
796,
357,
2395,
48209,
258,
85,
13033,
7,
77,
8,
764,
10,
352,
8,
764,
9,
2124,
2475,
14,
17,
1303,
2580,
48209,
258,
85,
2173,
319,
657,
492,
87,
2475,
198,
81,
796,
352,
13,
15,
1303,
826,
18645,
1988,
357,
9464,
318,
9672,
284,
307,
6632,
8,
198,
198,
50,
796,
10437,
14106,
7,
15,
492,
19,
9,
87,
2475,
8,
198,
198,
84,
158,
224,
222,
7,
87,
8,
796,
379,
272,
7,
87,
27493,
7,
46582,
1343,
379,
272,
7,
87,
12,
24,
8,
1343,
379,
272,
7,
20,
12,
87,
4008,
14,
46582,
61,
17,
532,
374,
1303,
34128,
416,
4600,
81,
63,
284,
3488,
6644,
786,
262,
826,
11843,
198,
220,
220,
7110,
7,
87,
18747,
11,
334,
158,
224,
222,
8,
198,
85,
796,
334,
158,
224,
222,
12195,
87,
18747,
8,
1303,
3815,
284,
779,
287,
15830,
198,
198,
76,
796,
2659,
7,
77,
11,
17,
8,
1303,
285,
9959,
299,
329,
3218,
5612,
198,
198,
2,
13610,
257,
26669,
6327,
68,
17593,
416,
22232,
262,
4308,
379,
262,
10706,
25,
198,
2,
775,
4155,
262,
4308,
45104,
262,
18645,
3403,
416,
1262,
198,
2,
262,
12784,
11188,
284,
1391,
6966,
7,
87,
828,
8615,
7,
18,
87,
828,
8615,
7,
20,
87,
828,
2644,
27422,
198,
53,
796,
1976,
27498,
7,
77,
11,
285,
8,
198,
1640,
479,
796,
352,
25,
76,
198,
220,
220,
569,
58,
45299,
74,
60,
796,
11138,
7,
50,
17414,
9107,
418,
7,
17,
9,
74,
12,
16,
1776,
16,
35944,
7,
87,
18747,
8,
198,
437,
198,
198,
66,
158,
224,
222,
796,
1976,
27498,
7,
17,
9,
76,
8,
198,
66,
158,
224,
222,
58,
17,
25,
17,
25,
437,
60,
796,
569,
59,
85,
1303,
466,
262,
4197,
198,
198,
69,
796,
11138,
7,
50,
11,
269,
158,
224,
222,
8,
1303,
45974,
407,
257,
922,
40874,
2233,
284,
18645,
19936,
84,
871,
11,
475,
1029,
19998,
481,
10921,
2952,
6949,
198,
220,
220,
7110,
26933,
87,
18747,
13,
10,
940,
26,
2124,
18747,
26,
2124,
18747,
7874,
940,
4357,
277,
11,
2124,
2475,
82,
16193,
12,
20,
11,
1315,
4008,
198,
220,
220,
41058,
0,
7,
87,
18747,
11,
334,
158,
224,
222,
11,
19736,
1096,
28,
15,
13,
17,
8,
198,
198,
43,
796,
9626,
452,
876,
7,
50,
11,
362,
8,
198,
220,
220,
406,
796,
24936,
7,
43,
58,
16,
25,
17,
76,
11,
16,
25,
17,
76,
12962,
198,
198,
31,
805,
541,
5039,
329,
309,
41888,
9107,
418,
7,
20,
1776,
657,
13,
15,
25,
15,
13,
486,
25,
16,
60,
198,
220,
220,
334,
62,
83,
796,
11138,
7,
50,
11,
1033,
7,
51,
9,
43,
27493,
66,
158,
224,
222,
8,
1343,
374,
1303,
15284,
4610,
290,
751,
736,
3488,
6644,
5612,
3381,
198,
220,
220,
7110,
32590,
15,
25,
15,
13,
2713,
25,
940,
11,
334,
62,
83,
11,
331,
2475,
82,
16193,
15,
11,
16,
828,
8177,
28,
25,
23108,
8,
198,
437,
198,
198,
31,
27908,
329,
309,
41888,
9107,
418,
7,
20,
1776,
657,
13,
15,
25,
15,
13,
20,
25,
1120,
60,
198,
220,
220,
334,
62,
83,
796,
11138,
7,
50,
11,
1033,
7,
51,
9,
43,
27493,
66,
158,
224,
222,
8,
1343,
374,
1303,
15284,
4610,
290,
751,
736,
3488,
6644,
5612,
3381,
198,
220,
220,
7110,
32590,
15,
25,
15,
13,
2713,
25,
940,
11,
334,
62,
83,
11,
331,
2475,
82,
16193,
15,
11,
16,
828,
8177,
28,
25,
23108,
8,
198,
437,
198
] | 2.531479 | 683 |
struct MinDomainVariableSelection{TakeObjective} <: AbstractVariableSelection{TakeObjective} end
MinDomainVariableSelection(;take_objective=true) = MinDomainVariableSelection{take_objective}()
function (::MinDomainVariableSelection{false})(cpmodel::CPModel)
selectedVar = nothing
minSize = typemax(Int)
for (k, x) in branchable_variables(cpmodel)
if x !== cpmodel.objective && !isbound(x) && length(x.domain) < minSize
selectedVar = x
minSize = length(x.domain)
end
end
if isnothing(selectedVar) && !isbound(cpmodel.objective)
return cpmodel.objective
end
return selectedVar
end
function (::MinDomainVariableSelection{true})(cpmodel::CPModel)
selectedVar = nothing
minSize = typemax(Int)
for (k, x) in branchable_variables(cpmodel)
if !isbound(x) && length(x.domain) < minSize
selectedVar = x
minSize = length(x.domain)
end
end
return selectedVar
end
| [
7249,
1855,
43961,
43015,
4653,
1564,
90,
12322,
10267,
425,
92,
1279,
25,
27741,
43015,
4653,
1564,
90,
12322,
10267,
425,
92,
886,
201,
198,
201,
198,
9452,
43961,
43015,
4653,
1564,
7,
26,
20657,
62,
15252,
425,
28,
7942,
8,
796,
1855,
43961,
43015,
4653,
1564,
90,
20657,
62,
15252,
425,
92,
3419,
201,
198,
201,
198,
8818,
357,
3712,
9452,
43961,
43015,
4653,
1564,
90,
9562,
92,
5769,
66,
4426,
375,
417,
3712,
8697,
17633,
8,
201,
198,
220,
220,
220,
6163,
19852,
796,
2147,
201,
198,
220,
220,
220,
949,
10699,
796,
2170,
368,
897,
7,
5317,
8,
201,
198,
220,
220,
220,
329,
357,
74,
11,
2124,
8,
287,
8478,
540,
62,
25641,
2977,
7,
66,
4426,
375,
417,
8,
201,
198,
220,
220,
220,
220,
220,
220,
220,
611,
2124,
5145,
855,
269,
4426,
375,
417,
13,
15252,
425,
11405,
5145,
271,
7784,
7,
87,
8,
11405,
4129,
7,
87,
13,
27830,
8,
1279,
949,
10699,
201,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6163,
19852,
796,
2124,
201,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
949,
10699,
796,
4129,
7,
87,
13,
27830,
8,
201,
198,
220,
220,
220,
220,
220,
220,
220,
886,
201,
198,
220,
220,
220,
886,
201,
198,
220,
220,
220,
611,
318,
22366,
7,
34213,
19852,
8,
11405,
5145,
271,
7784,
7,
66,
4426,
375,
417,
13,
15252,
425,
8,
201,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
269,
4426,
375,
417,
13,
15252,
425,
201,
198,
220,
220,
220,
886,
201,
198,
220,
220,
220,
1441,
6163,
19852,
201,
198,
437,
201,
198,
201,
198,
8818,
357,
3712,
9452,
43961,
43015,
4653,
1564,
90,
7942,
92,
5769,
66,
4426,
375,
417,
3712,
8697,
17633,
8,
201,
198,
220,
220,
220,
6163,
19852,
796,
2147,
201,
198,
220,
220,
220,
949,
10699,
796,
2170,
368,
897,
7,
5317,
8,
201,
198,
220,
220,
220,
329,
357,
74,
11,
2124,
8,
287,
8478,
540,
62,
25641,
2977,
7,
66,
4426,
375,
417,
8,
201,
198,
220,
220,
220,
220,
220,
220,
220,
611,
5145,
271,
7784,
7,
87,
8,
11405,
4129,
7,
87,
13,
27830,
8,
1279,
949,
10699,
201,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6163,
19852,
796,
2124,
201,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
949,
10699,
796,
4129,
7,
87,
13,
27830,
8,
201,
198,
220,
220,
220,
220,
220,
220,
220,
886,
201,
198,
220,
220,
220,
886,
201,
198,
220,
220,
220,
1441,
6163,
19852,
201,
198,
437,
201,
198
] | 2.323462 | 439 |
using StatsFuns: logistic
using Plots
βs = [0, 0.2, 0.5, 0.8, 1.0, 1.2, 1.5, 2, 3, 5, 7, 8, 10, 20]
plot([x -> logistic(β * x) for β in βs],
-2, 2,
alpha = 0.8,
legend = :none,
ylims = [0, 1])
| [
3500,
20595,
37,
13271,
25,
2604,
2569,
198,
3500,
1345,
1747,
198,
198,
26638,
82,
796,
685,
15,
11,
657,
13,
17,
11,
657,
13,
20,
11,
657,
13,
23,
11,
352,
13,
15,
11,
352,
13,
17,
11,
352,
13,
20,
11,
362,
11,
513,
11,
642,
11,
767,
11,
807,
11,
838,
11,
1160,
60,
198,
198,
29487,
26933,
87,
4613,
2604,
2569,
7,
26638,
1635,
2124,
8,
329,
27169,
287,
27169,
82,
4357,
198,
220,
220,
220,
220,
220,
220,
220,
532,
17,
11,
362,
11,
198,
220,
220,
220,
220,
220,
220,
220,
17130,
796,
657,
13,
23,
11,
198,
220,
220,
220,
220,
220,
220,
220,
8177,
796,
1058,
23108,
11,
198,
220,
220,
220,
220,
220,
220,
220,
331,
2475,
82,
796,
685,
15,
11,
352,
12962,
198
] | 1.69403 | 134 |
function HHG(f::TVF) where {TVF<:AbstractVector{<:AbstractFloat}}
n = length(f)
spec = fft(f)
return abs.(spec) .^ 2 / n^2
end
function HHGfreq(f::TVF, ncyc) where {TVF<:AbstractVector{<:AbstractFloat}}
x = collect(range(1, length(f), length = length(f)))
return x ./ ncyc
end
| [
8818,
47138,
38,
7,
69,
3712,
6849,
37,
8,
810,
1391,
6849,
37,
27,
25,
23839,
38469,
90,
27,
25,
23839,
43879,
11709,
198,
220,
220,
220,
299,
796,
4129,
7,
69,
8,
198,
220,
220,
220,
1020,
796,
277,
701,
7,
69,
8,
198,
220,
220,
220,
1441,
2352,
12195,
16684,
8,
764,
61,
362,
1220,
299,
61,
17,
198,
437,
198,
8818,
47138,
38,
19503,
80,
7,
69,
3712,
6849,
37,
11,
299,
948,
66,
8,
810,
1391,
6849,
37,
27,
25,
23839,
38469,
90,
27,
25,
23839,
43879,
11709,
198,
220,
220,
220,
2124,
796,
2824,
7,
9521,
7,
16,
11,
4129,
7,
69,
828,
4129,
796,
4129,
7,
69,
22305,
198,
220,
220,
220,
1441,
2124,
24457,
299,
948,
66,
198,
437,
198
] | 2.338583 | 127 |
"Parameters for right-hand side function of implicit partitioned Runge-Kutta methods."
mutable struct ParametersIPRK{DT, TT, D, S, ET <: NamedTuple} <: Parameters{DT,TT}
equs::ET
tab::PartitionedTableau{TT}
Δt::TT
t::TT
q::Vector{DT}
p::Vector{DT}
function ParametersIPRK{DT,D}(equs::ET, tab::PartitionedTableau{TT}, Δt::TT) where {D, DT, TT, ET <: NamedTuple}
new{DT, TT, D, tab.s, ET}(equs, tab, Δt, zero(TT), zeros(DT,D), zeros(DT,D))
end
end
@doc raw"""
Implicit partitioned Runge-Kutta integrator cache.
### Fields
* `q̃`: initial guess of q
* `p̃`: initial guess of p
* `ṽ`: initial guess of v
* `f̃`: initial guess of f
* `s̃`: holds shift due to periodicity of solution
* `Q`: internal stages of q
* `P`: internal stages of p
* `V`: internal stages of v
* `F`: internal stages of f
* `Y`: vector field of internal stages of q
* `Z`: vector field of internal stages of p
"""
struct IntegratorCacheIPRK{ST,D,S} <: PODEIntegratorCache{ST,D}
q̃::Vector{ST}
p̃::Vector{ST}
ṽ::Vector{ST}
f̃::Vector{ST}
s̃::Vector{ST}
Q::Vector{Vector{ST}}
P::Vector{Vector{ST}}
V::Vector{Vector{ST}}
F::Vector{Vector{ST}}
Y::Vector{Vector{ST}}
Z::Vector{Vector{ST}}
function IntegratorCacheIPRK{ST,D,S}() where {ST,D,S}
# create temporary vectors
q̃ = zeros(ST,D)
p̃ = zeros(ST,D)
ṽ = zeros(ST,D)
f̃ = zeros(ST,D)
s̃ = zeros(ST,D)
# create internal stage vectors
Q = create_internal_stage_vector(ST, D, S)
P = create_internal_stage_vector(ST, D, S)
V = create_internal_stage_vector(ST, D, S)
F = create_internal_stage_vector(ST, D, S)
Y = create_internal_stage_vector(ST, D, S)
Z = create_internal_stage_vector(ST, D, S)
new(q̃, p̃, ṽ, f̃, s̃, Q, P, V, F, Y, Z)
end
end
function IntegratorCache(params::ParametersIPRK{DT,TT,D,S}; kwargs...) where {DT,TT,D,S}
IntegratorCacheIPRK{DT,D,S}(; kwargs...)
end
function IntegratorCache{ST}(params::ParametersIPRK{DT,TT,D,S}; kwargs...) where {ST,DT,TT,D,S}
IntegratorCacheIPRK{ST,D,S}(; kwargs...)
end
@inline CacheType(ST, params::ParametersIPRK{DT,TT,D,S}) where {DT,TT,D,S} = IntegratorCacheIPRK{ST,D,S}
@doc raw"""
Implicit partitioned Runge-Kutta integrator solving the system
```math
\begin{aligned}
V_{n,i} &= v (Q_{n,i}, P_{n,i}) , &
Q_{n,i} &= q_{n} + h \sum \limits_{j=1}^{s} a_{ij} \, V_{n,j} , &
q_{n+1} &= q_{n} + h \sum \limits_{i=1}^{s} b_{i} \, V_{n,i} , \\
F_{n,i} &= f (Q_{n,i}, P_{n,i}) , &
P_{n,i} &= p_{n} + h \sum \limits_{i=1}^{s} \bar{a}_{ij} \, F_{n,j} , &
p_{n+1} &= p_{n} + h \sum \limits_{i=1}^{s} \bar{b}_{i} \, F_{n,i} ,
\end{aligned}
```
Usually we are interested in Hamiltonian systems, where
```math
\begin{aligned}
V_{n,i} &= \dfrac{\partial H}{\partial p} (Q_{n,i}, P_{n,i}) , &
F_{n,i} &= - \dfrac{\partial H}{\partial q} (Q_{n,i}, P_{n,i}) ,
\end{aligned}
```
and tableaus satisfying the symplecticity conditions
```math
\begin{aligned}
b_{i} \bar{a}_{ij} + \bar{b}_{j} a_{ji} &= b_{i} \bar{b}_{j} , &
\bar{b}_i &= b_i .
\end{aligned}
```
"""
struct IntegratorIPRK{DT, TT, D, S, PT <: ParametersIPRK{DT,TT},
ST <: NonlinearSolver{DT},
IT <: InitialGuessPODE{TT}} <: AbstractIntegratorPRK{DT,TT}
params::PT
solver::ST
iguess::IT
caches::CacheDict{PT}
function IntegratorIPRK(params::ParametersIPRK{DT,TT,D,S}, solver::ST, iguess::IT, caches) where {DT,TT,D,S,ST,IT}
new{DT, TT, D, S, typeof(params), ST, IT}(params, solver, iguess, caches)
end
function IntegratorIPRK{DT,D}(equations::NamedTuple, tableau::PartitionedTableau{TT}, Δt::TT) where {DT,TT,D}
# get number of stages
S = tableau.s
# create params
params = ParametersIPRK{DT,D}(equations, tableau, Δt)
# create cache dict
caches = CacheDict(params)
# create solver
solver = create_nonlinear_solver(DT, 2*D*S, params, caches)
# create initial guess
iguess = InitialGuessPODE(get_config(:ig_extrapolation), equations[:v], equations[:f], Δt)
# create integrator
IntegratorIPRK(params, solver, iguess, caches)
end
function IntegratorIPRK{DT,D}(v::Function, f::Function, tableau::PartitionedTableau{TT}, Δt::TT; kwargs...) where {DT,TT,D}
IntegratorIPRK{DT,D}(NamedTuple{(:v,:f)}((v,f)), tableau, Δt; kwargs...)
end
function IntegratorIPRK{DT,D}(v::Function, f::Function, h::Function, tableau::PartitionedTableau{TT}, Δt::TT; kwargs...) where {DT,TT,D}
IntegratorIPRK{DT,D}(NamedTuple{(:v,:f,:h)}((v,f,h)), tableau, Δt; kwargs...)
end
function IntegratorIPRK(equation::Union{PODE{DT}, HODE{DT}}, tableau::PartitionedTableau{TT}, Δt::TT; kwargs...) where {DT,TT}
IntegratorIPRK{DT, ndims(equation)}(get_functions(equation), tableau, Δt; kwargs...)
end
end
@inline Base.ndims(::IntegratorIPRK{DT,TT,D,S}) where {DT,TT,D,S} = D
function initialize!(int::IntegratorIPRK, sol::AtomicSolutionPODE)
sol.t̄ = sol.t - timestep(int)
equations(int)[:v](sol.t, sol.q, sol.p, sol.v)
equations(int)[:f](sol.t, sol.q, sol.p, sol.f)
initialize!(int.iguess, sol.t, sol.q, sol.p, sol.v, sol.f,
sol.t̄, sol.q̄, sol.p̄, sol.v̄, sol.f̄)
end
function update_params!(int::IntegratorIPRK, sol::AtomicSolutionPODE)
# set time for nonlinear solver and copy previous solution
int.params.t = sol.t
int.params.q .= sol.q
int.params.p .= sol.p
end
function initial_guess!(int::IntegratorIPRK{DT}, sol::AtomicSolutionPODE{DT},
cache::IntegratorCacheIPRK{DT}=int.caches[DT]) where {DT}
for i in eachstage(int)
evaluate!(int.iguess, sol.q̄, sol.p̄, sol.v̄, sol.f̄,
sol.q, sol.p, sol.v, sol.f,
cache.Q[i], cache.P[i], cache.V[i], cache.F[i],
tableau(int).q.c[i], tableau(int).p.c[i])
end
for i in eachstage(int)
for k in eachdim(int)
int.solver.x[2*(ndims(int)*(i-1)+k-1)+1] = 0
int.solver.x[2*(ndims(int)*(i-1)+k-1)+2] = 0
for j in eachstage(int)
int.solver.x[2*(ndims(int)*(i-1)+k-1)+1] += tableau(int).q.a[i,j] * cache.V[j][k]
int.solver.x[2*(ndims(int)*(i-1)+k-1)+2] += tableau(int).p.a[i,j] * cache.F[j][k]
end
end
end
end
function compute_stages!(x::Vector{ST}, Q::Vector{Vector{ST}}, V::Vector{Vector{ST}}, Y::Vector{Vector{ST}},
P::Vector{Vector{ST}}, F::Vector{Vector{ST}}, Z::Vector{Vector{ST}},
params::ParametersIPRK{DT,TT,D,S}) where {ST,DT,TT,D,S}
local tqᵢ::TT
local tpᵢ::TT
for i in 1:S
for k in 1:D
# copy y to Y and Z
Y[i][k] = x[2*(D*(i-1)+k-1)+1]
Z[i][k] = x[2*(D*(i-1)+k-1)+2]
# compute Q and P
Q[i][k] = params.q[k] + params.Δt * Y[i][k]
P[i][k] = params.p[k] + params.Δt * Z[i][k]
end
# compute time of internal stage
tqᵢ = params.t + params.Δt * params.tab.q.c[i]
tpᵢ = params.t + params.Δt * params.tab.p.c[i]
# compute v(Q,P) and f(Q,P)
params.equs[:v](tqᵢ, Q[i], P[i], V[i])
params.equs[:f](tpᵢ, Q[i], P[i], F[i])
end
end
# Compute stages of implicit partitioned Runge-Kutta methods.
function function_stages!(x::Vector{ST}, b::Vector{ST}, params::ParametersIPRK{DT,TT,D,S},
caches::CacheDict) where {ST,DT,TT,D,S}
# get cache for internal stages
cache = caches[ST]
# compute stages from nonlinear solver solution x
compute_stages!(x, cache.Q, cache.V, cache.Y, cache.P, cache.F, cache.Z, params)
# compute b = - [(Y-AV), (Z-AF)]
for i in 1:S
for k in 1:D
b[2*(D*(i-1)+k-1)+1] = - cache.Y[i][k]
b[2*(D*(i-1)+k-1)+2] = - cache.Z[i][k]
for j in 1:S
b[2*(D*(i-1)+k-1)+1] += params.tab.q.a[i,j] * cache.V[j][k]
b[2*(D*(i-1)+k-1)+2] += params.tab.p.a[i,j] * cache.F[j][k]
end
end
end
end
function integrate_step!(int::IntegratorIPRK{DT,TT}, sol::AtomicSolutionPODE{DT,TT},
cache::IntegratorCacheIPRK{DT}=int.caches[DT]) where {DT,TT}
# update nonlinear solver parameters from atomic solution
update_params!(int, sol)
# compute initial guess
initial_guess!(int, sol, cache)
# reset atomic solution
reset!(sol, timestep(int))
# call nonlinear solver
solve!(int.solver)
# print solver status
print_solver_status(int.solver.status, int.solver.params)
# check if solution contains NaNs or error bounds are violated
check_solver_status(int.solver.status, int.solver.params)
# compute vector fields at internal stages
compute_stages!(int.solver.x, cache.Q, cache.V, cache.Y,
cache.P, cache.F, cache.Z, int.params)
# compute final update
update_solution!(sol.q, sol.q̃, cache.V, tableau(int).q.b, tableau(int).q.b̂, timestep(int))
update_solution!(sol.p, sol.p̃, cache.F, tableau(int).p.b, tableau(int).p.b̂, timestep(int))
# copy solution to initial guess
update_vector_fields!(int.iguess, sol.t, sol.q, sol.p, sol.v, sol.f)
end
| [
198,
1,
48944,
329,
826,
12,
4993,
1735,
2163,
286,
16992,
18398,
276,
5660,
469,
12,
42,
315,
8326,
5050,
526,
198,
76,
18187,
2878,
40117,
4061,
49,
42,
90,
24544,
11,
26653,
11,
360,
11,
311,
11,
12152,
1279,
25,
34441,
51,
29291,
92,
1279,
25,
40117,
90,
24544,
11,
15751,
92,
198,
220,
220,
220,
37430,
385,
3712,
2767,
198,
220,
220,
220,
7400,
3712,
7841,
653,
276,
10962,
559,
90,
15751,
92,
198,
220,
220,
220,
37455,
83,
3712,
15751,
628,
220,
220,
220,
256,
3712,
15751,
198,
220,
220,
220,
10662,
3712,
38469,
90,
24544,
92,
198,
220,
220,
220,
279,
3712,
38469,
90,
24544,
92,
628,
220,
220,
220,
2163,
40117,
4061,
49,
42,
90,
24544,
11,
35,
92,
7,
27363,
385,
3712,
2767,
11,
7400,
3712,
7841,
653,
276,
10962,
559,
90,
15751,
5512,
37455,
83,
3712,
15751,
8,
810,
1391,
35,
11,
24311,
11,
26653,
11,
12152,
1279,
25,
34441,
51,
29291,
92,
198,
220,
220,
220,
220,
220,
220,
220,
649,
90,
24544,
11,
26653,
11,
360,
11,
7400,
13,
82,
11,
12152,
92,
7,
27363,
385,
11,
7400,
11,
37455,
83,
11,
6632,
7,
15751,
828,
1976,
27498,
7,
24544,
11,
35,
828,
1976,
27498,
7,
24544,
11,
35,
4008,
198,
220,
220,
220,
886,
198,
437,
628,
198,
31,
15390,
8246,
37811,
198,
29710,
3628,
18398,
276,
5660,
469,
12,
42,
315,
8326,
4132,
12392,
12940,
13,
198,
198,
21017,
23948,
198,
198,
9,
4600,
80,
136,
225,
63,
25,
4238,
4724,
286,
10662,
198,
9,
4600,
79,
136,
225,
63,
25,
4238,
4724,
286,
279,
198,
9,
4600,
85,
136,
225,
63,
25,
4238,
4724,
286,
410,
198,
9,
4600,
69,
136,
225,
63,
25,
4238,
4724,
286,
277,
198,
9,
4600,
82,
136,
225,
63,
25,
6622,
6482,
2233,
284,
2278,
8467,
286,
4610,
198,
9,
4600,
48,
63,
25,
5387,
9539,
286,
10662,
198,
9,
4600,
47,
63,
25,
5387,
9539,
286,
279,
198,
9,
4600,
53,
63,
25,
5387,
9539,
286,
410,
198,
9,
4600,
37,
63,
25,
5387,
9539,
286,
277,
198,
9,
4600,
56,
63,
25,
15879,
2214,
286,
5387,
9539,
286,
10662,
198,
9,
4600,
57,
63,
25,
15879,
2214,
286,
5387,
9539,
286,
279,
198,
37811,
198,
7249,
15995,
12392,
30562,
4061,
49,
42,
90,
2257,
11,
35,
11,
50,
92,
1279,
25,
350,
16820,
34500,
12392,
30562,
90,
2257,
11,
35,
92,
198,
220,
220,
220,
10662,
136,
225,
3712,
38469,
90,
2257,
92,
198,
220,
220,
220,
279,
136,
225,
3712,
38469,
90,
2257,
92,
198,
220,
220,
220,
410,
136,
225,
3712,
38469,
90,
2257,
92,
198,
220,
220,
220,
277,
136,
225,
3712,
38469,
90,
2257,
92,
198,
220,
220,
220,
264,
136,
225,
3712,
38469,
90,
2257,
92,
628,
220,
220,
220,
1195,
3712,
38469,
90,
38469,
90,
2257,
11709,
198,
220,
220,
220,
350,
3712,
38469,
90,
38469,
90,
2257,
11709,
198,
220,
220,
220,
569,
3712,
38469,
90,
38469,
90,
2257,
11709,
198,
220,
220,
220,
376,
3712,
38469,
90,
38469,
90,
2257,
11709,
198,
220,
220,
220,
575,
3712,
38469,
90,
38469,
90,
2257,
11709,
198,
220,
220,
220,
1168,
3712,
38469,
90,
38469,
90,
2257,
11709,
628,
220,
220,
220,
2163,
15995,
12392,
30562,
4061,
49,
42,
90,
2257,
11,
35,
11,
50,
92,
3419,
810,
1391,
2257,
11,
35,
11,
50,
92,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
2251,
8584,
30104,
198,
220,
220,
220,
220,
220,
220,
220,
10662,
136,
225,
796,
1976,
27498,
7,
2257,
11,
35,
8,
198,
220,
220,
220,
220,
220,
220,
220,
279,
136,
225,
796,
1976,
27498,
7,
2257,
11,
35,
8,
198,
220,
220,
220,
220,
220,
220,
220,
410,
136,
225,
796,
1976,
27498,
7,
2257,
11,
35,
8,
198,
220,
220,
220,
220,
220,
220,
220,
277,
136,
225,
796,
1976,
27498,
7,
2257,
11,
35,
8,
198,
220,
220,
220,
220,
220,
220,
220,
264,
136,
225,
796,
1976,
27498,
7,
2257,
11,
35,
8,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
2251,
5387,
3800,
30104,
198,
220,
220,
220,
220,
220,
220,
220,
1195,
796,
2251,
62,
32538,
62,
14247,
62,
31364,
7,
2257,
11,
360,
11,
311,
8,
198,
220,
220,
220,
220,
220,
220,
220,
350,
796,
2251,
62,
32538,
62,
14247,
62,
31364,
7,
2257,
11,
360,
11,
311,
8,
198,
220,
220,
220,
220,
220,
220,
220,
569,
796,
2251,
62,
32538,
62,
14247,
62,
31364,
7,
2257,
11,
360,
11,
311,
8,
198,
220,
220,
220,
220,
220,
220,
220,
376,
796,
2251,
62,
32538,
62,
14247,
62,
31364,
7,
2257,
11,
360,
11,
311,
8,
198,
220,
220,
220,
220,
220,
220,
220,
575,
796,
2251,
62,
32538,
62,
14247,
62,
31364,
7,
2257,
11,
360,
11,
311,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1168,
796,
2251,
62,
32538,
62,
14247,
62,
31364,
7,
2257,
11,
360,
11,
311,
8,
628,
220,
220,
220,
220,
220,
220,
220,
649,
7,
80,
136,
225,
11,
279,
136,
225,
11,
410,
136,
225,
11,
277,
136,
225,
11,
264,
136,
225,
11,
1195,
11,
350,
11,
569,
11,
376,
11,
575,
11,
1168,
8,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
15995,
12392,
30562,
7,
37266,
3712,
48944,
4061,
49,
42,
90,
24544,
11,
15751,
11,
35,
11,
50,
19629,
479,
86,
22046,
23029,
810,
1391,
24544,
11,
15751,
11,
35,
11,
50,
92,
198,
220,
220,
220,
15995,
12392,
30562,
4061,
49,
42,
90,
24544,
11,
35,
11,
50,
92,
7,
26,
479,
86,
22046,
23029,
198,
437,
198,
198,
8818,
15995,
12392,
30562,
90,
2257,
92,
7,
37266,
3712,
48944,
4061,
49,
42,
90,
24544,
11,
15751,
11,
35,
11,
50,
19629,
479,
86,
22046,
23029,
810,
1391,
2257,
11,
24544,
11,
15751,
11,
35,
11,
50,
92,
198,
220,
220,
220,
15995,
12392,
30562,
4061,
49,
42,
90,
2257,
11,
35,
11,
50,
92,
7,
26,
479,
86,
22046,
23029,
198,
437,
198,
198,
31,
45145,
34088,
6030,
7,
2257,
11,
42287,
3712,
48944,
4061,
49,
42,
90,
24544,
11,
15751,
11,
35,
11,
50,
30072,
810,
1391,
24544,
11,
15751,
11,
35,
11,
50,
92,
796,
15995,
12392,
30562,
4061,
49,
42,
90,
2257,
11,
35,
11,
50,
92,
628,
198,
31,
15390,
8246,
37811,
198,
29710,
3628,
18398,
276,
5660,
469,
12,
42,
315,
8326,
4132,
12392,
18120,
262,
1080,
198,
15506,
63,
11018,
198,
59,
27471,
90,
41634,
92,
198,
53,
23330,
77,
11,
72,
92,
1222,
28,
410,
357,
48,
23330,
77,
11,
72,
5512,
350,
23330,
77,
11,
72,
30072,
837,
1222,
198,
48,
23330,
77,
11,
72,
92,
1222,
28,
10662,
23330,
77,
92,
1343,
289,
3467,
16345,
3467,
49196,
23330,
73,
28,
16,
92,
36796,
82,
92,
257,
23330,
2926,
92,
3467,
11,
569,
23330,
77,
11,
73,
92,
837,
1222,
198,
80,
23330,
77,
10,
16,
92,
1222,
28,
10662,
23330,
77,
92,
1343,
289,
3467,
16345,
3467,
49196,
23330,
72,
28,
16,
92,
36796,
82,
92,
275,
23330,
72,
92,
3467,
11,
569,
23330,
77,
11,
72,
92,
837,
26867,
198,
37,
23330,
77,
11,
72,
92,
1222,
28,
277,
357,
48,
23330,
77,
11,
72,
5512,
350,
23330,
77,
11,
72,
30072,
837,
1222,
198,
47,
23330,
77,
11,
72,
92,
1222,
28,
279,
23330,
77,
92,
1343,
289,
220,
3467,
16345,
3467,
49196,
23330,
72,
28,
16,
92,
36796,
82,
92,
3467,
5657,
90,
64,
92,
23330,
2926,
92,
3467,
11,
376,
23330,
77,
11,
73,
92,
837,
1222,
198,
79,
23330,
77,
10,
16,
92,
1222,
28,
279,
23330,
77,
92,
1343,
289,
3467,
16345,
3467,
49196,
23330,
72,
28,
16,
92,
36796,
82,
92,
3467,
5657,
90,
65,
92,
23330,
72,
92,
3467,
11,
376,
23330,
77,
11,
72,
92,
837,
198,
59,
437,
90,
41634,
92,
198,
15506,
63,
198,
37887,
356,
389,
4609,
287,
11582,
666,
3341,
11,
810,
198,
15506,
63,
11018,
198,
59,
27471,
90,
41634,
92,
198,
53,
23330,
77,
11,
72,
92,
1222,
28,
3467,
7568,
11510,
31478,
47172,
367,
18477,
59,
47172,
279,
92,
357,
48,
23330,
77,
11,
72,
5512,
350,
23330,
77,
11,
72,
30072,
837,
1222,
198,
37,
23330,
77,
11,
72,
92,
1222,
28,
532,
3467,
7568,
11510,
31478,
47172,
367,
18477,
59,
47172,
10662,
92,
357,
48,
23330,
77,
11,
72,
5512,
350,
23330,
77,
11,
72,
30072,
837,
220,
198,
59,
437,
90,
41634,
92,
198,
15506,
63,
198,
392,
3084,
8717,
19201,
262,
10558,
801,
8467,
3403,
198,
15506,
63,
11018,
198,
59,
27471,
90,
41634,
92,
198,
65,
23330,
72,
92,
3467,
5657,
90,
64,
92,
23330,
2926,
92,
1343,
3467,
5657,
90,
65,
92,
23330,
73,
92,
257,
23330,
7285,
92,
1222,
28,
275,
23330,
72,
92,
3467,
5657,
90,
65,
92,
23330,
73,
92,
837,
1222,
198,
59,
5657,
90,
65,
92,
62,
72,
1222,
28,
275,
62,
72,
764,
198,
59,
437,
90,
41634,
92,
198,
15506,
63,
198,
37811,
198,
7249,
15995,
12392,
4061,
49,
42,
90,
24544,
11,
26653,
11,
360,
11,
311,
11,
19310,
1279,
25,
40117,
4061,
49,
42,
90,
24544,
11,
15751,
5512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3563,
1279,
25,
8504,
29127,
50,
14375,
90,
24544,
5512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7283,
1279,
25,
20768,
8205,
408,
47,
16820,
90,
15751,
11709,
1279,
25,
27741,
34500,
12392,
4805,
42,
90,
24544,
11,
15751,
92,
198,
220,
220,
220,
42287,
3712,
11571,
198,
220,
220,
220,
1540,
332,
3712,
2257,
198,
220,
220,
220,
45329,
84,
408,
3712,
2043,
198,
220,
220,
220,
50177,
3712,
30562,
35,
713,
90,
11571,
92,
628,
220,
220,
220,
2163,
15995,
12392,
4061,
49,
42,
7,
37266,
3712,
48944,
4061,
49,
42,
90,
24544,
11,
15751,
11,
35,
11,
50,
5512,
1540,
332,
3712,
2257,
11,
45329,
84,
408,
3712,
2043,
11,
50177,
8,
810,
1391,
24544,
11,
15751,
11,
35,
11,
50,
11,
2257,
11,
2043,
92,
198,
220,
220,
220,
220,
220,
220,
220,
649,
90,
24544,
11,
26653,
11,
360,
11,
311,
11,
2099,
1659,
7,
37266,
828,
3563,
11,
7283,
92,
7,
37266,
11,
1540,
332,
11,
45329,
84,
408,
11,
50177,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2163,
15995,
12392,
4061,
49,
42,
90,
24544,
11,
35,
92,
7,
4853,
602,
3712,
45,
2434,
51,
29291,
11,
3084,
559,
3712,
7841,
653,
276,
10962,
559,
90,
15751,
5512,
37455,
83,
3712,
15751,
8,
810,
1391,
24544,
11,
15751,
11,
35,
92,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
651,
1271,
286,
9539,
198,
220,
220,
220,
220,
220,
220,
220,
311,
796,
3084,
559,
13,
82,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
2251,
42287,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
796,
40117,
4061,
49,
42,
90,
24544,
11,
35,
92,
7,
4853,
602,
11,
3084,
559,
11,
37455,
83,
8,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
2251,
12940,
8633,
198,
220,
220,
220,
220,
220,
220,
220,
50177,
796,
34088,
35,
713,
7,
37266,
8,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
2251,
1540,
332,
198,
220,
220,
220,
220,
220,
220,
220,
1540,
332,
796,
2251,
62,
13159,
29127,
62,
82,
14375,
7,
24544,
11,
362,
9,
35,
9,
50,
11,
42287,
11,
50177,
8,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
2251,
4238,
4724,
198,
220,
220,
220,
220,
220,
220,
220,
45329,
84,
408,
796,
20768,
8205,
408,
47,
16820,
7,
1136,
62,
11250,
7,
25,
328,
62,
2302,
2416,
21417,
828,
27490,
58,
25,
85,
4357,
27490,
58,
25,
69,
4357,
37455,
83,
8,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
2251,
4132,
12392,
198,
220,
220,
220,
220,
220,
220,
220,
15995,
12392,
4061,
49,
42,
7,
37266,
11,
1540,
332,
11,
45329,
84,
408,
11,
50177,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2163,
15995,
12392,
4061,
49,
42,
90,
24544,
11,
35,
92,
7,
85,
3712,
22203,
11,
277,
3712,
22203,
11,
3084,
559,
3712,
7841,
653,
276,
10962,
559,
90,
15751,
5512,
37455,
83,
3712,
15751,
26,
479,
86,
22046,
23029,
810,
1391,
24544,
11,
15751,
11,
35,
92,
198,
220,
220,
220,
220,
220,
220,
220,
15995,
12392,
4061,
49,
42,
90,
24544,
11,
35,
92,
7,
45,
2434,
51,
29291,
90,
7,
25,
85,
11,
25,
69,
38165,
19510,
85,
11,
69,
36911,
3084,
559,
11,
37455,
83,
26,
479,
86,
22046,
23029,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2163,
15995,
12392,
4061,
49,
42,
90,
24544,
11,
35,
92,
7,
85,
3712,
22203,
11,
277,
3712,
22203,
11,
289,
3712,
22203,
11,
3084,
559,
3712,
7841,
653,
276,
10962,
559,
90,
15751,
5512,
37455,
83,
3712,
15751,
26,
479,
86,
22046,
23029,
810,
1391,
24544,
11,
15751,
11,
35,
92,
198,
220,
220,
220,
220,
220,
220,
220,
15995,
12392,
4061,
49,
42,
90,
24544,
11,
35,
92,
7,
45,
2434,
51,
29291,
90,
7,
25,
85,
11,
25,
69,
11,
25,
71,
38165,
19510,
85,
11,
69,
11,
71,
36911,
3084,
559,
11,
37455,
83,
26,
479,
86,
22046,
23029,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2163,
15995,
12392,
4061,
49,
42,
7,
4853,
341,
3712,
38176,
90,
47,
16820,
90,
24544,
5512,
367,
16820,
90,
24544,
92,
5512,
3084,
559,
3712,
7841,
653,
276,
10962,
559,
90,
15751,
5512,
37455,
83,
3712,
15751,
26,
479,
86,
22046,
23029,
810,
1391,
24544,
11,
15751,
92,
198,
220,
220,
220,
220,
220,
220,
220,
15995,
12392,
4061,
49,
42,
90,
24544,
11,
299,
67,
12078,
7,
4853,
341,
38165,
7,
1136,
62,
12543,
2733,
7,
4853,
341,
828,
3084,
559,
11,
37455,
83,
26,
479,
86,
22046,
23029,
198,
220,
220,
220,
886,
198,
437,
628,
198,
31,
45145,
7308,
13,
358,
12078,
7,
3712,
34500,
12392,
4061,
49,
42,
90,
24544,
11,
15751,
11,
35,
11,
50,
30072,
810,
1391,
24544,
11,
15751,
11,
35,
11,
50,
92,
796,
360,
628,
198,
8818,
41216,
0,
7,
600,
3712,
34500,
12392,
4061,
49,
42,
11,
1540,
3712,
2953,
10179,
46344,
47,
16820,
8,
198,
220,
220,
220,
1540,
13,
83,
136,
226,
796,
1540,
13,
83,
532,
4628,
395,
538,
7,
600,
8,
628,
220,
220,
220,
27490,
7,
600,
38381,
25,
85,
16151,
34453,
13,
83,
11,
1540,
13,
80,
11,
1540,
13,
79,
11,
1540,
13,
85,
8,
198,
220,
220,
220,
27490,
7,
600,
38381,
25,
69,
16151,
34453,
13,
83,
11,
1540,
13,
80,
11,
1540,
13,
79,
11,
1540,
13,
69,
8,
628,
220,
220,
220,
41216,
0,
7,
600,
13,
328,
84,
408,
11,
1540,
13,
83,
11,
1540,
13,
80,
11,
1540,
13,
79,
11,
1540,
13,
85,
11,
1540,
13,
69,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1540,
13,
83,
136,
226,
11,
1540,
13,
80,
136,
226,
11,
1540,
13,
79,
136,
226,
11,
1540,
13,
85,
136,
226,
11,
1540,
13,
69,
136,
226,
8,
198,
437,
628,
198,
8818,
4296,
62,
37266,
0,
7,
600,
3712,
34500,
12392,
4061,
49,
42,
11,
1540,
3712,
2953,
10179,
46344,
47,
16820,
8,
198,
220,
220,
220,
1303,
900,
640,
329,
1729,
29127,
1540,
332,
290,
4866,
2180,
4610,
198,
220,
220,
220,
493,
13,
37266,
13,
83,
220,
796,
1540,
13,
83,
198,
220,
220,
220,
493,
13,
37266,
13,
80,
764,
28,
1540,
13,
80,
198,
220,
220,
220,
493,
13,
37266,
13,
79,
764,
28,
1540,
13,
79,
198,
437,
628,
198,
8818,
4238,
62,
5162,
408,
0,
7,
600,
3712,
34500,
12392,
4061,
49,
42,
90,
24544,
5512,
1540,
3712,
2953,
10179,
46344,
47,
16820,
90,
24544,
5512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
12940,
3712,
34500,
12392,
30562,
4061,
49,
42,
90,
24544,
92,
28,
600,
13,
66,
3694,
58,
24544,
12962,
810,
1391,
24544,
92,
628,
220,
220,
220,
329,
1312,
287,
1123,
14247,
7,
600,
8,
198,
220,
220,
220,
220,
220,
220,
220,
13446,
0,
7,
600,
13,
328,
84,
408,
11,
1540,
13,
80,
136,
226,
11,
1540,
13,
79,
136,
226,
11,
1540,
13,
85,
136,
226,
11,
1540,
13,
69,
136,
226,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1540,
13,
80,
11,
1540,
13,
79,
11,
1540,
13,
85,
11,
1540,
13,
69,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
12940,
13,
48,
58,
72,
4357,
12940,
13,
47,
58,
72,
4357,
12940,
13,
53,
58,
72,
4357,
12940,
13,
37,
58,
72,
4357,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3084,
559,
7,
600,
737,
80,
13,
66,
58,
72,
4357,
3084,
559,
7,
600,
737,
79,
13,
66,
58,
72,
12962,
198,
220,
220,
220,
886,
198,
220,
220,
220,
329,
1312,
287,
1123,
14247,
7,
600,
8,
198,
220,
220,
220,
220,
220,
220,
220,
329,
479,
287,
1123,
27740,
7,
600,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
493,
13,
82,
14375,
13,
87,
58,
17,
9,
7,
358,
12078,
7,
600,
27493,
7,
72,
12,
16,
47762,
74,
12,
16,
47762,
16,
60,
796,
657,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
493,
13,
82,
14375,
13,
87,
58,
17,
9,
7,
358,
12078,
7,
600,
27493,
7,
72,
12,
16,
47762,
74,
12,
16,
47762,
17,
60,
796,
657,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
329,
474,
287,
1123,
14247,
7,
600,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
493,
13,
82,
14375,
13,
87,
58,
17,
9,
7,
358,
12078,
7,
600,
27493,
7,
72,
12,
16,
47762,
74,
12,
16,
47762,
16,
60,
15853,
3084,
559,
7,
600,
737,
80,
13,
64,
58,
72,
11,
73,
60,
1635,
12940,
13,
53,
58,
73,
7131,
74,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
493,
13,
82,
14375,
13,
87,
58,
17,
9,
7,
358,
12078,
7,
600,
27493,
7,
72,
12,
16,
47762,
74,
12,
16,
47762,
17,
60,
15853,
3084,
559,
7,
600,
737,
79,
13,
64,
58,
72,
11,
73,
60,
1635,
12940,
13,
37,
58,
73,
7131,
74,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
437,
628,
198,
8818,
24061,
62,
301,
1095,
0,
7,
87,
3712,
38469,
90,
2257,
5512,
1195,
3712,
38469,
90,
38469,
90,
2257,
92,
5512,
569,
3712,
38469,
90,
38469,
90,
2257,
92,
5512,
575,
3712,
38469,
90,
38469,
90,
2257,
92,
5512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
350,
3712,
38469,
90,
38469,
90,
2257,
92,
5512,
376,
3712,
38469,
90,
38469,
90,
2257,
92,
5512,
1168,
3712,
38469,
90,
38469,
90,
2257,
92,
5512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
42287,
3712,
48944,
4061,
49,
42,
90,
24544,
11,
15751,
11,
35,
11,
50,
30072,
810,
1391,
2257,
11,
24544,
11,
15751,
11,
35,
11,
50,
92,
198,
220,
220,
220,
1957,
256,
80,
39611,
95,
3712,
15751,
198,
220,
220,
220,
1957,
256,
79,
39611,
95,
3712,
15751,
628,
220,
220,
220,
329,
1312,
287,
352,
25,
50,
198,
220,
220,
220,
220,
220,
220,
220,
329,
479,
287,
352,
25,
35,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
4866,
331,
284,
575,
290,
1168,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
575,
58,
72,
7131,
74,
60,
796,
2124,
58,
17,
9,
7,
35,
9,
7,
72,
12,
16,
47762,
74,
12,
16,
47762,
16,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1168,
58,
72,
7131,
74,
60,
796,
2124,
58,
17,
9,
7,
35,
9,
7,
72,
12,
16,
47762,
74,
12,
16,
47762,
17,
60,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
24061,
1195,
290,
350,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1195,
58,
72,
7131,
74,
60,
796,
42287,
13,
80,
58,
74,
60,
1343,
42287,
13,
138,
242,
83,
1635,
575,
58,
72,
7131,
74,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
350,
58,
72,
7131,
74,
60,
796,
42287,
13,
79,
58,
74,
60,
1343,
42287,
13,
138,
242,
83,
1635,
1168,
58,
72,
7131,
74,
60,
198,
220,
220,
220,
220,
220,
220,
220,
886,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
24061,
640,
286,
5387,
3800,
198,
220,
220,
220,
220,
220,
220,
220,
256,
80,
39611,
95,
796,
42287,
13,
83,
1343,
42287,
13,
138,
242,
83,
1635,
42287,
13,
8658,
13,
80,
13,
66,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
256,
79,
39611,
95,
796,
42287,
13,
83,
1343,
42287,
13,
138,
242,
83,
1635,
42287,
13,
8658,
13,
79,
13,
66,
58,
72,
60,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
24061,
410,
7,
48,
11,
47,
8,
290,
277,
7,
48,
11,
47,
8,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
13,
27363,
385,
58,
25,
85,
16151,
83,
80,
39611,
95,
11,
1195,
58,
72,
4357,
350,
58,
72,
4357,
569,
58,
72,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
13,
27363,
385,
58,
25,
69,
16151,
34788,
39611,
95,
11,
1195,
58,
72,
4357,
350,
58,
72,
4357,
376,
58,
72,
12962,
198,
220,
220,
220,
886,
198,
437,
628,
198,
2,
3082,
1133,
9539,
286,
16992,
18398,
276,
5660,
469,
12,
42,
315,
8326,
5050,
13,
198,
8818,
2163,
62,
301,
1095,
0,
7,
87,
3712,
38469,
90,
2257,
5512,
275,
3712,
38469,
90,
2257,
5512,
42287,
3712,
48944,
4061,
49,
42,
90,
24544,
11,
15751,
11,
35,
11,
50,
5512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
50177,
3712,
30562,
35,
713,
8,
810,
1391,
2257,
11,
24544,
11,
15751,
11,
35,
11,
50,
92,
628,
220,
220,
220,
1303,
651,
12940,
329,
5387,
9539,
198,
220,
220,
220,
12940,
796,
50177,
58,
2257,
60,
628,
220,
220,
220,
1303,
24061,
9539,
422,
1729,
29127,
1540,
332,
4610,
2124,
198,
220,
220,
220,
24061,
62,
301,
1095,
0,
7,
87,
11,
12940,
13,
48,
11,
12940,
13,
53,
11,
12940,
13,
56,
11,
12940,
13,
47,
11,
12940,
13,
37,
11,
12940,
13,
57,
11,
42287,
8,
628,
220,
220,
220,
1303,
24061,
275,
796,
532,
47527,
56,
12,
10116,
828,
357,
57,
12,
8579,
15437,
198,
220,
220,
220,
329,
1312,
287,
352,
25,
50,
198,
220,
220,
220,
220,
220,
220,
220,
329,
479,
287,
352,
25,
35,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
275,
58,
17,
9,
7,
35,
9,
7,
72,
12,
16,
47762,
74,
12,
16,
47762,
16,
60,
796,
532,
12940,
13,
56,
58,
72,
7131,
74,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
275,
58,
17,
9,
7,
35,
9,
7,
72,
12,
16,
47762,
74,
12,
16,
47762,
17,
60,
796,
532,
12940,
13,
57,
58,
72,
7131,
74,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
329,
474,
287,
352,
25,
50,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
275,
58,
17,
9,
7,
35,
9,
7,
72,
12,
16,
47762,
74,
12,
16,
47762,
16,
60,
15853,
42287,
13,
8658,
13,
80,
13,
64,
58,
72,
11,
73,
60,
1635,
12940,
13,
53,
58,
73,
7131,
74,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
275,
58,
17,
9,
7,
35,
9,
7,
72,
12,
16,
47762,
74,
12,
16,
47762,
17,
60,
15853,
42287,
13,
8658,
13,
79,
13,
64,
58,
72,
11,
73,
60,
1635,
12940,
13,
37,
58,
73,
7131,
74,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
437,
628,
198,
8818,
19386,
62,
9662,
0,
7,
600,
3712,
34500,
12392,
4061,
49,
42,
90,
24544,
11,
15751,
5512,
1540,
3712,
2953,
10179,
46344,
47,
16820,
90,
24544,
11,
15751,
5512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
12940,
3712,
34500,
12392,
30562,
4061,
49,
42,
90,
24544,
92,
28,
600,
13,
66,
3694,
58,
24544,
12962,
810,
1391,
24544,
11,
15751,
92,
198,
220,
220,
220,
1303,
4296,
1729,
29127,
1540,
332,
10007,
422,
17226,
4610,
198,
220,
220,
220,
4296,
62,
37266,
0,
7,
600,
11,
1540,
8,
628,
220,
220,
220,
1303,
24061,
4238,
4724,
198,
220,
220,
220,
4238,
62,
5162,
408,
0,
7,
600,
11,
1540,
11,
12940,
8,
628,
220,
220,
220,
1303,
13259,
17226,
4610,
198,
220,
220,
220,
13259,
0,
7,
34453,
11,
4628,
395,
538,
7,
600,
4008,
628,
220,
220,
220,
1303,
869,
1729,
29127,
1540,
332,
198,
220,
220,
220,
8494,
0,
7,
600,
13,
82,
14375,
8,
628,
220,
220,
220,
1303,
3601,
1540,
332,
3722,
198,
220,
220,
220,
3601,
62,
82,
14375,
62,
13376,
7,
600,
13,
82,
14375,
13,
13376,
11,
493,
13,
82,
14375,
13,
37266,
8,
628,
220,
220,
220,
1303,
2198,
611,
4610,
4909,
11013,
47503,
393,
4049,
22303,
389,
13998,
198,
220,
220,
220,
2198,
62,
82,
14375,
62,
13376,
7,
600,
13,
82,
14375,
13,
13376,
11,
493,
13,
82,
14375,
13,
37266,
8,
628,
220,
220,
220,
1303,
24061,
15879,
7032,
379,
5387,
9539,
198,
220,
220,
220,
24061,
62,
301,
1095,
0,
7,
600,
13,
82,
14375,
13,
87,
11,
12940,
13,
48,
11,
12940,
13,
53,
11,
12940,
13,
56,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
12940,
13,
47,
11,
12940,
13,
37,
11,
12940,
13,
57,
11,
493,
13,
37266,
8,
628,
220,
220,
220,
1303,
24061,
2457,
4296,
198,
220,
220,
220,
4296,
62,
82,
2122,
0,
7,
34453,
13,
80,
11,
1540,
13,
80,
136,
225,
11,
12940,
13,
53,
11,
3084,
559,
7,
600,
737,
80,
13,
65,
11,
3084,
559,
7,
600,
737,
80,
13,
65,
136,
224,
11,
4628,
395,
538,
7,
600,
4008,
198,
220,
220,
220,
4296,
62,
82,
2122,
0,
7,
34453,
13,
79,
11,
1540,
13,
79,
136,
225,
11,
12940,
13,
37,
11,
3084,
559,
7,
600,
737,
79,
13,
65,
11,
3084,
559,
7,
600,
737,
79,
13,
65,
136,
224,
11,
4628,
395,
538,
7,
600,
4008,
628,
220,
220,
220,
1303,
4866,
4610,
284,
4238,
4724,
198,
220,
220,
220,
4296,
62,
31364,
62,
25747,
0,
7,
600,
13,
328,
84,
408,
11,
1540,
13,
83,
11,
1540,
13,
80,
11,
1540,
13,
79,
11,
1540,
13,
85,
11,
1540,
13,
69,
8,
198,
437,
198
] | 1.977662 | 4,790 |
abstract type AbstractTableauSplitting{T <: Real} <: AbstractTableau{T} end
function get_splitting_coefficients(r, a::Vector{T}, b::Vector{T}) where {T}
@assert length(a) == length(b)
s = length(a)
f = zeros(Int, 2r*s)
c = zeros(T, 2r*s)
for i in 1:s
for j in 1:r
f[(2i-2)*r+j] = j
c[(2i-2)*r+j] = a[i]
end
for j in 1:r
f[(2i-1)*r+j] = r-j+1
c[(2i-1)*r+j] = b[i]
end
end
return f, c
end
@doc raw"""
Tableau for general splitting methods for vector fields with two components A and B.
Integrator:
```math
\varphi_{\tau} = \varphi_{b_s \tau}^{B} \circ \varphi_{a_s \tau}^{A} \circ \dotsc \circ \varphi_{b_1 \tau}^{B} \circ \varphi_{a_1 \tau}^{A}
```
"""
struct TableauSplitting{T} <: AbstractTableauSplitting{T}
@HeaderTableau
a::Vector{T}
b::Vector{T}
function TableauSplitting{T}(name, o, s, a, b) where {T}
@assert s == length(a) == length(b)
new(name, o, s, a, b)
end
end
function TableauSplitting(name, o, a::Vector{T}, b::Vector{T}) where {T}
TableauSplitting{T}(name, o, length(a), a, b)
end
function get_splitting_coefficients(nequs, tableau::TableauSplitting{T}) where {T}
@assert nequs == 2
@assert length(tableau.a) == length(tableau.b)
s = length(tableau.a)
f = zeros(Int, 2s)
c = zeros(T, 2s)
for i in eachindex(tableau.a, tableau.b)
f[2*(i-1)+1] = 1
c[2*(i-1)+1] = tableau.a[i]
f[2*(i-1)+2] = 2
c[2*(i-1)+2] = tableau.b[i]
end
# select all entries with non-vanishing coefficients
y = c .!= 0
return f[y], c[y]
end
@doc raw"""
Tableau for non-symmetric splitting methods.
See McLachlan, Quispel, 2003, Equ. (4.10).
The methods A and B are the composition of all vector fields in the SODE
and its adjoint, respectively.
Basic method: Lie composition
```math
\begin{aligned}
\varphi_{\tau}^{A} &= \varphi_{\tau}^{v_1} \circ \varphi_{\tau}^{v_2} \circ \dotsc \circ \varphi_{\tau}^{v_{r-1}} \circ \varphi_{\tau}^{v_r} \\
\varphi_{\tau}^{B} &= \varphi_{\tau}^{v_r} \circ \varphi_{\tau}^{v_{r-1}} \circ \dotsc \circ \varphi_{\tau}^{v_2} \circ \varphi_{\tau}^{v_1}
\end{aligned}
```
Integrator:
```math
\varphi_{\tau}^{NS} = \varphi_{b_s \tau}^{B} \circ \varphi_{a_s \tau}^{A} \circ \dotsc \circ \varphi_{b_1 \tau}^{B} \circ \varphi_{a_1 \tau}^{A}
```
"""
struct TableauSplittingNS{T} <: AbstractTableauSplitting{T}
@HeaderTableau
a::Vector{T}
b::Vector{T}
function TableauSplittingNS{T}(name, o, s, a, b) where {T}
@assert s == length(a) == length(b)
new(name, o, s, a, b)
end
end
function TableauSplittingNS(name, o, a::Vector{T}, b::Vector{T}) where {T}
TableauSplittingNS{T}(name, o, length(a), a, b)
end
function get_splitting_coefficients(nequs, tableau::TableauSplittingNS)
# R = length(equation.v)
# S = tableau.s
#
# f = zeros(Int, 2R*S)
# c = zeros(TT, 2R*S)
#
# for i in 1:S
# for j in 1:R
# f[(2i-2)*R+j] = j
# c[(2i-2)*R+j] = tableau.a[i]
# end
# for j in 1:R
# f[(2i-1)*R+j] = R-j+1
# c[(2i-1)*R+j] = tableau.b[i]
# end
# end
get_splitting_coefficients(nequs, tableau.a, tableau.b)
end
@doc raw"""
Tableau for symmetric splitting methods with general stages.
See McLachlan, Quispel, 2003, Equ. (4.11).
Basic method: Lie composition
```math
\begin{aligned}
\varphi_{\tau}^{A} &= \varphi_{\tau}^{v_1} \circ \varphi_{\tau}^{v_2} \circ \dotsc \circ \varphi_{\tau}^{v_{r-1}} \circ \varphi_{\tau}^{v_r} \\
\varphi_{\tau}^{B} &= \varphi_{\tau}^{v_r} \circ \varphi_{\tau}^{v_{r-1}} \circ \dotsc \circ \varphi_{\tau}^{v_2} \circ \varphi_{\tau}^{v_1}
\end{aligned}
```
Integrator:
```math
\varphi_{\tau}^{GS} = \varphi_{a_1 \tau}^{A} \circ \varphi_{b_1 \tau}^{B} \circ \dotsc \circ \varphi_{b_1 \tau}^{B} \circ \varphi_{a_1 \tau}^{A}
```
"""
struct TableauSplittingGS{T} <: AbstractTableauSplitting{T}
@HeaderTableau
a::Vector{T}
b::Vector{T}
function TableauSplittingGS{T}(name, o, s, a, b) where {T}
@assert s == length(a) == length(b)
new(name, o, s, a, b)
end
end
function TableauSplittingGS(name, o, a::Vector{T}, b::Vector{T}) where {T}
TableauSplittingGS{T}(name, o, length(a), a, b)
end
function get_splitting_coefficients(nequs, tableau::TableauSplittingGS)
# R = nequs
# S = tableau.s
#
# f = zeros(Int, 2R*S)
# c = zeros(TT, 2R*S)
#
# for i in 1:S
# for j in 1:R
# f[(2i-2)*R+j] = j
# c[(2i-2)*R+j] = tableau.a[i]
# end
# for j in R:-1:1
# f[(2i-1)*R+j] = R-j+1
# c[(2i-1)*R+j] = tableau.b[i]
# end
# end
f, c = get_splitting_coefficients(nequs, tableau.a, tableau.b)
vcat(f, f[end:-1:1]), vcat(c, c[end:-1:1])
end
@doc raw"""
Tableau for symmetric splitting methods with symmetric stages.
See McLachlan, Quispel, 2003, Equ. (4.6).
Basic method: symmetric Strang composition
```math
\varphi_{\tau}^{A} = \varphi_{\tau/2}^{v_1} \circ \varphi_{\tau/2}^{v_2} \circ \dotsc \circ \varphi_{\tau/2}^{v_{r-1}} \circ \varphi_{\tau/2}^{v_r} \circ \varphi_{\tau/2}^{v_r} \circ \varphi_{\tau/2}^{v_{r-1}} \circ \dotsc \circ \varphi_{\tau/2}^{v_2} \circ \varphi_{\tau/2}^{v_1}
```
Integrator:
```math
\varphi_{\tau}^{SS} = \varphi_{a_1 \tau}^{A} \circ \varphi_{a_2 \tau}^{A} \circ \dotsc \circ \varphi_{a_s \tau}^{A} \circ \dotsc \circ \varphi_{a_2 \tau}^{A} \circ \varphi_{a_1 \tau}^{A}
```
"""
struct TableauSplittingSS{T} <: AbstractTableauSplitting{T}
@HeaderTableau
a::Vector{T}
function TableauSplittingSS{T}(name, o, s, a) where {T}
@assert s == length(a)
new(name, o, s, a)
end
end
function TableauSplittingSS(name, o, a::Vector{T}) where {T}
TableauSplittingSS{T}(name, o, length(a), a)
end
function get_splitting_coefficients(nequs, tableau::TableauSplittingSS{T}) where {T}
r = nequs
a = vcat(tableau.a, tableau.a[end-1:-1:1]) ./ 2
s = length(a)
f = zeros(Int, 2r*s)
c = zeros(T, 2r*s)
for i in 1:s
for j in 1:r
f[(2i-2)*r+j] = j
c[(2i-2)*r+j] = a[i]
f[(2i-1)*r+j] = r-j+1
c[(2i-1)*r+j] = a[i]
end
end
f, c
end
| [
198,
397,
8709,
2099,
27741,
10962,
559,
26568,
2535,
90,
51,
1279,
25,
6416,
92,
1279,
25,
27741,
10962,
559,
90,
51,
92,
886,
198,
198,
8818,
651,
62,
22018,
2535,
62,
1073,
41945,
7,
81,
11,
257,
3712,
38469,
90,
51,
5512,
275,
3712,
38469,
90,
51,
30072,
810,
1391,
51,
92,
198,
220,
220,
220,
2488,
30493,
4129,
7,
64,
8,
6624,
4129,
7,
65,
8,
628,
220,
220,
220,
264,
796,
4129,
7,
64,
8,
198,
220,
220,
220,
277,
796,
1976,
27498,
7,
5317,
11,
362,
81,
9,
82,
8,
198,
220,
220,
220,
269,
796,
1976,
27498,
7,
51,
11,
220,
220,
362,
81,
9,
82,
8,
628,
220,
220,
220,
329,
1312,
287,
352,
25,
82,
198,
220,
220,
220,
220,
220,
220,
220,
329,
474,
287,
352,
25,
81,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
277,
58,
7,
17,
72,
12,
17,
27493,
81,
10,
73,
60,
796,
474,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
58,
7,
17,
72,
12,
17,
27493,
81,
10,
73,
60,
796,
257,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
329,
474,
287,
352,
25,
81,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
277,
58,
7,
17,
72,
12,
16,
27493,
81,
10,
73,
60,
796,
374,
12,
73,
10,
16,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
58,
7,
17,
72,
12,
16,
27493,
81,
10,
73,
60,
796,
275,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1441,
277,
11,
269,
198,
437,
628,
198,
31,
15390,
8246,
37811,
198,
10962,
559,
329,
2276,
26021,
5050,
329,
15879,
7032,
351,
734,
6805,
317,
290,
347,
13,
198,
198,
34500,
12392,
25,
198,
15506,
63,
11018,
198,
59,
7785,
34846,
23330,
59,
83,
559,
92,
796,
3467,
7785,
34846,
23330,
65,
62,
82,
3467,
83,
559,
92,
36796,
33,
92,
3467,
21170,
3467,
7785,
34846,
23330,
64,
62,
82,
3467,
83,
559,
92,
36796,
32,
92,
3467,
21170,
3467,
26518,
1416,
3467,
21170,
3467,
7785,
34846,
23330,
65,
62,
16,
3467,
83,
559,
92,
36796,
33,
92,
3467,
21170,
3467,
7785,
34846,
23330,
64,
62,
16,
3467,
83,
559,
92,
36796,
32,
92,
198,
15506,
63,
198,
37811,
198,
7249,
8655,
559,
26568,
2535,
90,
51,
92,
1279,
25,
27741,
10962,
559,
26568,
2535,
90,
51,
92,
198,
220,
220,
220,
2488,
39681,
10962,
559,
628,
220,
220,
220,
257,
3712,
38469,
90,
51,
92,
198,
220,
220,
220,
275,
3712,
38469,
90,
51,
92,
628,
220,
220,
220,
2163,
8655,
559,
26568,
2535,
90,
51,
92,
7,
3672,
11,
267,
11,
264,
11,
257,
11,
275,
8,
810,
1391,
51,
92,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
30493,
264,
6624,
4129,
7,
64,
8,
6624,
4129,
7,
65,
8,
198,
220,
220,
220,
220,
220,
220,
220,
649,
7,
3672,
11,
267,
11,
264,
11,
257,
11,
275,
8,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
8655,
559,
26568,
2535,
7,
3672,
11,
267,
11,
257,
3712,
38469,
90,
51,
5512,
275,
3712,
38469,
90,
51,
30072,
810,
1391,
51,
92,
198,
220,
220,
220,
8655,
559,
26568,
2535,
90,
51,
92,
7,
3672,
11,
267,
11,
4129,
7,
64,
828,
257,
11,
275,
8,
198,
437,
198,
198,
8818,
651,
62,
22018,
2535,
62,
1073,
41945,
7,
710,
45260,
11,
3084,
559,
3712,
10962,
559,
26568,
2535,
90,
51,
30072,
810,
1391,
51,
92,
198,
220,
220,
220,
2488,
30493,
497,
45260,
6624,
362,
198,
220,
220,
220,
2488,
30493,
4129,
7,
11487,
559,
13,
64,
8,
6624,
4129,
7,
11487,
559,
13,
65,
8,
628,
220,
220,
220,
264,
796,
4129,
7,
11487,
559,
13,
64,
8,
198,
220,
220,
220,
277,
796,
1976,
27498,
7,
5317,
11,
362,
82,
8,
198,
220,
220,
220,
269,
796,
1976,
27498,
7,
51,
11,
220,
220,
362,
82,
8,
628,
220,
220,
220,
329,
1312,
287,
1123,
9630,
7,
11487,
559,
13,
64,
11,
3084,
559,
13,
65,
8,
198,
220,
220,
220,
220,
220,
220,
220,
277,
58,
17,
9,
7,
72,
12,
16,
47762,
16,
60,
796,
352,
198,
220,
220,
220,
220,
220,
220,
220,
269,
58,
17,
9,
7,
72,
12,
16,
47762,
16,
60,
796,
3084,
559,
13,
64,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
277,
58,
17,
9,
7,
72,
12,
16,
47762,
17,
60,
796,
362,
198,
220,
220,
220,
220,
220,
220,
220,
269,
58,
17,
9,
7,
72,
12,
16,
47762,
17,
60,
796,
3084,
559,
13,
65,
58,
72,
60,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
2922,
477,
12784,
351,
1729,
12,
10438,
3929,
44036,
198,
220,
220,
220,
331,
796,
269,
764,
0,
28,
657,
628,
220,
220,
220,
1441,
277,
58,
88,
4357,
269,
58,
88,
60,
198,
437,
628,
198,
31,
15390,
8246,
37811,
198,
10962,
559,
329,
1729,
12,
1837,
3020,
19482,
26021,
5050,
13,
198,
220,
220,
220,
4091,
18365,
620,
9620,
11,
2264,
8802,
417,
11,
5816,
11,
7889,
13,
357,
19,
13,
940,
737,
198,
220,
220,
220,
383,
5050,
317,
290,
347,
389,
262,
11742,
286,
477,
15879,
7032,
287,
262,
311,
16820,
198,
220,
220,
220,
290,
663,
9224,
1563,
11,
8148,
13,
198,
198,
26416,
2446,
25,
12060,
11742,
198,
15506,
63,
11018,
198,
59,
27471,
90,
41634,
92,
198,
59,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
32,
92,
1222,
28,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
62,
16,
92,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
62,
17,
92,
3467,
21170,
3467,
26518,
1416,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
23330,
81,
12,
16,
11709,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
62,
81,
92,
26867,
198,
59,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
33,
92,
1222,
28,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
62,
81,
92,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
23330,
81,
12,
16,
11709,
3467,
21170,
3467,
26518,
1416,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
62,
17,
92,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
62,
16,
92,
198,
59,
437,
90,
41634,
92,
198,
15506,
63,
198,
198,
34500,
12392,
25,
198,
15506,
63,
11018,
198,
59,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
8035,
92,
796,
3467,
7785,
34846,
23330,
65,
62,
82,
3467,
83,
559,
92,
36796,
33,
92,
3467,
21170,
3467,
7785,
34846,
23330,
64,
62,
82,
3467,
83,
559,
92,
36796,
32,
92,
3467,
21170,
3467,
26518,
1416,
3467,
21170,
3467,
7785,
34846,
23330,
65,
62,
16,
3467,
83,
559,
92,
36796,
33,
92,
3467,
21170,
3467,
7785,
34846,
23330,
64,
62,
16,
3467,
83,
559,
92,
36796,
32,
92,
198,
15506,
63,
198,
37811,
198,
7249,
8655,
559,
26568,
2535,
8035,
90,
51,
92,
1279,
25,
27741,
10962,
559,
26568,
2535,
90,
51,
92,
198,
220,
220,
220,
2488,
39681,
10962,
559,
628,
220,
220,
220,
257,
3712,
38469,
90,
51,
92,
198,
220,
220,
220,
275,
3712,
38469,
90,
51,
92,
628,
220,
220,
220,
2163,
8655,
559,
26568,
2535,
8035,
90,
51,
92,
7,
3672,
11,
267,
11,
264,
11,
257,
11,
275,
8,
810,
1391,
51,
92,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
30493,
264,
6624,
4129,
7,
64,
8,
6624,
4129,
7,
65,
8,
198,
220,
220,
220,
220,
220,
220,
220,
649,
7,
3672,
11,
267,
11,
264,
11,
257,
11,
275,
8,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
8655,
559,
26568,
2535,
8035,
7,
3672,
11,
267,
11,
257,
3712,
38469,
90,
51,
5512,
275,
3712,
38469,
90,
51,
30072,
810,
1391,
51,
92,
198,
220,
220,
220,
8655,
559,
26568,
2535,
8035,
90,
51,
92,
7,
3672,
11,
267,
11,
4129,
7,
64,
828,
257,
11,
275,
8,
198,
437,
198,
198,
8818,
651,
62,
22018,
2535,
62,
1073,
41945,
7,
710,
45260,
11,
3084,
559,
3712,
10962,
559,
26568,
2535,
8035,
8,
198,
220,
220,
220,
1303,
371,
796,
4129,
7,
4853,
341,
13,
85,
8,
198,
220,
220,
220,
1303,
311,
796,
3084,
559,
13,
82,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
277,
796,
1976,
27498,
7,
5317,
11,
362,
49,
9,
50,
8,
198,
220,
220,
220,
1303,
269,
796,
1976,
27498,
7,
15751,
11,
220,
362,
49,
9,
50,
8,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
329,
1312,
287,
352,
25,
50,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
329,
474,
287,
352,
25,
49,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
220,
220,
220,
220,
277,
58,
7,
17,
72,
12,
17,
27493,
49,
10,
73,
60,
796,
474,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
220,
220,
220,
220,
269,
58,
7,
17,
72,
12,
17,
27493,
49,
10,
73,
60,
796,
3084,
559,
13,
64,
58,
72,
60,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
886,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
329,
474,
287,
352,
25,
49,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
220,
220,
220,
220,
277,
58,
7,
17,
72,
12,
16,
27493,
49,
10,
73,
60,
796,
371,
12,
73,
10,
16,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
220,
220,
220,
220,
269,
58,
7,
17,
72,
12,
16,
27493,
49,
10,
73,
60,
796,
3084,
559,
13,
65,
58,
72,
60,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
886,
198,
220,
220,
220,
1303,
886,
628,
220,
220,
220,
651,
62,
22018,
2535,
62,
1073,
41945,
7,
710,
45260,
11,
3084,
559,
13,
64,
11,
3084,
559,
13,
65,
8,
198,
437,
628,
198,
31,
15390,
8246,
37811,
198,
10962,
559,
329,
23606,
19482,
26021,
5050,
351,
2276,
9539,
13,
198,
220,
220,
220,
4091,
18365,
620,
9620,
11,
2264,
8802,
417,
11,
5816,
11,
7889,
13,
357,
19,
13,
1157,
737,
198,
198,
26416,
2446,
25,
12060,
11742,
198,
15506,
63,
11018,
198,
59,
27471,
90,
41634,
92,
198,
59,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
32,
92,
1222,
28,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
62,
16,
92,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
62,
17,
92,
3467,
21170,
3467,
26518,
1416,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
23330,
81,
12,
16,
11709,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
62,
81,
92,
26867,
198,
59,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
33,
92,
1222,
28,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
62,
81,
92,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
23330,
81,
12,
16,
11709,
3467,
21170,
3467,
26518,
1416,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
62,
17,
92,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
85,
62,
16,
92,
198,
59,
437,
90,
41634,
92,
198,
15506,
63,
198,
198,
34500,
12392,
25,
198,
15506,
63,
11018,
198,
59,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
14313,
92,
796,
3467,
7785,
34846,
23330,
64,
62,
16,
3467,
83,
559,
92,
36796,
32,
92,
3467,
21170,
3467,
7785,
34846,
23330,
65,
62,
16,
3467,
83,
559,
92,
36796,
33,
92,
3467,
21170,
3467,
26518,
1416,
3467,
21170,
3467,
7785,
34846,
23330,
65,
62,
16,
3467,
83,
559,
92,
36796,
33,
92,
3467,
21170,
3467,
7785,
34846,
23330,
64,
62,
16,
3467,
83,
559,
92,
36796,
32,
92,
198,
15506,
63,
198,
37811,
198,
7249,
8655,
559,
26568,
2535,
14313,
90,
51,
92,
1279,
25,
27741,
10962,
559,
26568,
2535,
90,
51,
92,
198,
220,
220,
220,
2488,
39681,
10962,
559,
628,
220,
220,
220,
257,
3712,
38469,
90,
51,
92,
198,
220,
220,
220,
275,
3712,
38469,
90,
51,
92,
628,
220,
220,
220,
2163,
8655,
559,
26568,
2535,
14313,
90,
51,
92,
7,
3672,
11,
267,
11,
264,
11,
257,
11,
275,
8,
810,
1391,
51,
92,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
30493,
264,
6624,
4129,
7,
64,
8,
6624,
4129,
7,
65,
8,
198,
220,
220,
220,
220,
220,
220,
220,
649,
7,
3672,
11,
267,
11,
264,
11,
257,
11,
275,
8,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
8655,
559,
26568,
2535,
14313,
7,
3672,
11,
267,
11,
257,
3712,
38469,
90,
51,
5512,
275,
3712,
38469,
90,
51,
30072,
810,
1391,
51,
92,
198,
220,
220,
220,
8655,
559,
26568,
2535,
14313,
90,
51,
92,
7,
3672,
11,
267,
11,
4129,
7,
64,
828,
257,
11,
275,
8,
198,
437,
198,
198,
8818,
651,
62,
22018,
2535,
62,
1073,
41945,
7,
710,
45260,
11,
3084,
559,
3712,
10962,
559,
26568,
2535,
14313,
8,
220,
198,
220,
220,
220,
1303,
371,
796,
497,
45260,
198,
220,
220,
220,
1303,
311,
796,
3084,
559,
13,
82,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
277,
796,
1976,
27498,
7,
5317,
11,
362,
49,
9,
50,
8,
198,
220,
220,
220,
1303,
269,
796,
1976,
27498,
7,
15751,
11,
220,
362,
49,
9,
50,
8,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
329,
1312,
287,
352,
25,
50,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
329,
474,
287,
352,
25,
49,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
220,
220,
220,
220,
277,
58,
7,
17,
72,
12,
17,
27493,
49,
10,
73,
60,
796,
474,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
220,
220,
220,
220,
269,
58,
7,
17,
72,
12,
17,
27493,
49,
10,
73,
60,
796,
3084,
559,
13,
64,
58,
72,
60,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
886,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
329,
474,
287,
371,
21912,
16,
25,
16,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
220,
220,
220,
220,
277,
58,
7,
17,
72,
12,
16,
27493,
49,
10,
73,
60,
796,
371,
12,
73,
10,
16,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
220,
220,
220,
220,
269,
58,
7,
17,
72,
12,
16,
27493,
49,
10,
73,
60,
796,
3084,
559,
13,
65,
58,
72,
60,
198,
220,
220,
220,
1303,
220,
220,
220,
220,
886,
198,
220,
220,
220,
1303,
886,
628,
220,
220,
220,
277,
11,
269,
796,
651,
62,
22018,
2535,
62,
1073,
41945,
7,
710,
45260,
11,
3084,
559,
13,
64,
11,
3084,
559,
13,
65,
8,
198,
220,
220,
220,
410,
9246,
7,
69,
11,
277,
58,
437,
21912,
16,
25,
16,
46570,
410,
9246,
7,
66,
11,
269,
58,
437,
21912,
16,
25,
16,
12962,
198,
437,
628,
198,
31,
15390,
8246,
37811,
198,
10962,
559,
329,
23606,
19482,
26021,
5050,
351,
23606,
19482,
9539,
13,
198,
220,
220,
220,
4091,
18365,
620,
9620,
11,
2264,
8802,
417,
11,
5816,
11,
7889,
13,
357,
19,
13,
21,
737,
198,
198,
26416,
2446,
25,
23606,
19482,
4285,
648,
11742,
198,
15506,
63,
11018,
198,
59,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
32,
92,
796,
3467,
7785,
34846,
23330,
59,
83,
559,
14,
17,
92,
36796,
85,
62,
16,
92,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
14,
17,
92,
36796,
85,
62,
17,
92,
3467,
21170,
3467,
26518,
1416,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
14,
17,
92,
36796,
85,
23330,
81,
12,
16,
11709,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
14,
17,
92,
36796,
85,
62,
81,
92,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
14,
17,
92,
36796,
85,
62,
81,
92,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
14,
17,
92,
36796,
85,
23330,
81,
12,
16,
11709,
3467,
21170,
3467,
26518,
1416,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
14,
17,
92,
36796,
85,
62,
17,
92,
3467,
21170,
3467,
7785,
34846,
23330,
59,
83,
559,
14,
17,
92,
36796,
85,
62,
16,
92,
198,
15506,
63,
198,
198,
34500,
12392,
25,
198,
15506,
63,
11018,
198,
59,
7785,
34846,
23330,
59,
83,
559,
92,
36796,
5432,
92,
796,
3467,
7785,
34846,
23330,
64,
62,
16,
3467,
83,
559,
92,
36796,
32,
92,
3467,
21170,
3467,
7785,
34846,
23330,
64,
62,
17,
3467,
83,
559,
92,
36796,
32,
92,
3467,
21170,
3467,
26518,
1416,
3467,
21170,
3467,
7785,
34846,
23330,
64,
62,
82,
3467,
83,
559,
92,
36796,
32,
92,
3467,
21170,
3467,
26518,
1416,
3467,
21170,
3467,
7785,
34846,
23330,
64,
62,
17,
3467,
83,
559,
92,
36796,
32,
92,
3467,
21170,
3467,
7785,
34846,
23330,
64,
62,
16,
3467,
83,
559,
92,
36796,
32,
92,
198,
15506,
63,
198,
37811,
198,
7249,
8655,
559,
26568,
2535,
5432,
90,
51,
92,
1279,
25,
27741,
10962,
559,
26568,
2535,
90,
51,
92,
198,
220,
220,
220,
2488,
39681,
10962,
559,
628,
220,
220,
220,
257,
3712,
38469,
90,
51,
92,
628,
220,
220,
220,
2163,
8655,
559,
26568,
2535,
5432,
90,
51,
92,
7,
3672,
11,
267,
11,
264,
11,
257,
8,
810,
1391,
51,
92,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
30493,
264,
6624,
4129,
7,
64,
8,
198,
220,
220,
220,
220,
220,
220,
220,
649,
7,
3672,
11,
267,
11,
264,
11,
257,
8,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
8655,
559,
26568,
2535,
5432,
7,
3672,
11,
267,
11,
257,
3712,
38469,
90,
51,
30072,
810,
1391,
51,
92,
198,
220,
220,
220,
8655,
559,
26568,
2535,
5432,
90,
51,
92,
7,
3672,
11,
267,
11,
4129,
7,
64,
828,
257,
8,
198,
437,
198,
198,
8818,
651,
62,
22018,
2535,
62,
1073,
41945,
7,
710,
45260,
11,
3084,
559,
3712,
10962,
559,
26568,
2535,
5432,
90,
51,
30072,
810,
1391,
51,
92,
198,
220,
220,
220,
374,
796,
497,
45260,
198,
220,
220,
220,
257,
796,
410,
9246,
7,
11487,
559,
13,
64,
11,
3084,
559,
13,
64,
58,
437,
12,
16,
21912,
16,
25,
16,
12962,
24457,
362,
198,
220,
220,
220,
264,
796,
4129,
7,
64,
8,
628,
220,
220,
220,
277,
796,
1976,
27498,
7,
5317,
11,
362,
81,
9,
82,
8,
198,
220,
220,
220,
269,
796,
1976,
27498,
7,
51,
11,
220,
220,
362,
81,
9,
82,
8,
628,
220,
220,
220,
329,
1312,
287,
352,
25,
82,
198,
220,
220,
220,
220,
220,
220,
220,
329,
474,
287,
352,
25,
81,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
277,
58,
7,
17,
72,
12,
17,
27493,
81,
10,
73,
60,
796,
474,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
58,
7,
17,
72,
12,
17,
27493,
81,
10,
73,
60,
796,
257,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
277,
58,
7,
17,
72,
12,
16,
27493,
81,
10,
73,
60,
796,
374,
12,
73,
10,
16,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
58,
7,
17,
72,
12,
16,
27493,
81,
10,
73,
60,
796,
257,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
277,
11,
269,
198,
437,
198
] | 1.934994 | 3,292 |
t = Template(; user=me)
pkg_dir = joinpath(t.dir, test_pkg)
@testset "AppVeyor" begin
@testset "Plugin creation" begin
p = AppVeyor()
@test isempty(p.gitignore)
@test p.src == joinpath(PkgTemplates.DEFAULTS_DIR, "appveyor.yml")
@test p.dest == ".appveyor.yml"
@test p.badges == [
Badge(
"Build Status",
"https://ci.appveyor.com/api/projects/status/github/{{USER}}/{{PKGNAME}}.jl?svg=true",
"https://ci.appveyor.com/project/{{USER}}/{{PKGNAME}}-jl",
),
]
@test isempty(p.view)
p = AppVeyor(; config_file=nothing)
@test p.src === nothing
p = AppVeyor(; config_file=test_file)
@test p.src == test_file
@test_throws ArgumentError AppVeyor(; config_file=fake_path)
end
@testset "Badge generation" begin
p = AppVeyor()
@test badges(p, me, test_pkg) == ["[](https://ci.appveyor.com/project/$me/$test_pkg-jl)"]
end
@testset "File generation" begin
# Without a coverage plugin in the template, there should be no post-test step.
p = AppVeyor()
@test gen_plugin(p, t, test_pkg) == [".appveyor.yml"]
@test isfile(joinpath(pkg_dir, ".appveyor.yml"))
appveyor = read(joinpath(pkg_dir, ".appveyor.yml"), String)
@test !occursin("on_success", appveyor)
@test !occursin("%JL_CODECOV_SCRIPT%", appveyor)
rm(joinpath(pkg_dir, ".appveyor.yml"))
# Generating the plugin with Codecov in the template should create a post-test step.
t.plugins[Codecov] = Codecov()
gen_plugin(p, t, test_pkg)
delete!(t.plugins, Codecov)
appveyor = read(joinpath(pkg_dir, ".appveyor.yml"), String)
@test occursin("on_success", appveyor)
@test occursin("%JL_CODECOV_SCRIPT%", appveyor)
rm(joinpath(pkg_dir, ".appveyor.yml"))
# TODO: Add Coveralls tests when AppVeyor.jl supports it.
p = AppVeyor(; config_file=nothing)
@test isempty(gen_plugin(p, t, test_pkg))
@test !isfile(joinpath(pkg_dir, ".appveyor.yml"))
end
end
rm(pkg_dir; recursive=true)
| [
83,
796,
37350,
7,
26,
2836,
28,
1326,
8,
198,
35339,
62,
15908,
796,
4654,
6978,
7,
83,
13,
15908,
11,
1332,
62,
35339,
8,
198,
198,
31,
9288,
2617,
366,
4677,
53,
2959,
273,
1,
2221,
198,
220,
220,
220,
2488,
9288,
2617,
366,
37233,
6282,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
279,
796,
2034,
53,
2959,
273,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
318,
28920,
7,
79,
13,
18300,
46430,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
279,
13,
10677,
6624,
4654,
6978,
7,
47,
10025,
12966,
17041,
13,
7206,
7708,
35342,
62,
34720,
11,
366,
1324,
3304,
273,
13,
88,
4029,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
279,
13,
16520,
6624,
27071,
1324,
3304,
273,
13,
88,
4029,
1,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
279,
13,
14774,
3212,
6624,
685,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
44308,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
15580,
12678,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
5450,
1378,
979,
13,
1324,
3304,
273,
13,
785,
14,
15042,
14,
42068,
14,
13376,
14,
12567,
14,
27007,
29904,
11709,
14,
27007,
40492,
16630,
10067,
11709,
13,
20362,
30,
21370,
70,
28,
7942,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
5450,
1378,
979,
13,
1324,
3304,
273,
13,
785,
14,
16302,
14,
27007,
29904,
11709,
14,
27007,
40492,
16630,
10067,
11709,
12,
20362,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
10612,
198,
220,
220,
220,
220,
220,
220,
220,
2361,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
318,
28920,
7,
79,
13,
1177,
8,
198,
220,
220,
220,
220,
220,
220,
220,
279,
796,
2034,
53,
2959,
273,
7,
26,
4566,
62,
7753,
28,
22366,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
279,
13,
10677,
24844,
2147,
198,
220,
220,
220,
220,
220,
220,
220,
279,
796,
2034,
53,
2959,
273,
7,
26,
4566,
62,
7753,
28,
9288,
62,
7753,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
279,
13,
10677,
6624,
1332,
62,
7753,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
62,
400,
8516,
45751,
12331,
2034,
53,
2959,
273,
7,
26,
4566,
62,
7753,
28,
30706,
62,
6978,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
22069,
469,
5270,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
279,
796,
2034,
53,
2959,
273,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
37583,
7,
79,
11,
502,
11,
1332,
62,
35339,
8,
6624,
14631,
58,
0,
58,
15580,
12678,
16151,
5450,
1378,
979,
13,
1324,
3304,
273,
13,
785,
14,
15042,
14,
42068,
14,
13376,
14,
12567,
32624,
1326,
32624,
9288,
62,
35339,
13,
20362,
30,
21370,
70,
28,
7942,
15437,
7,
5450,
1378,
979,
13,
1324,
3304,
273,
13,
785,
14,
16302,
32624,
1326,
32624,
9288,
62,
35339,
12,
20362,
8,
8973,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
8979,
5270,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
9170,
257,
5197,
13877,
287,
262,
11055,
11,
612,
815,
307,
645,
1281,
12,
9288,
2239,
13,
198,
220,
220,
220,
220,
220,
220,
220,
279,
796,
2034,
53,
2959,
273,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2429,
62,
33803,
7,
79,
11,
256,
11,
1332,
62,
35339,
8,
6624,
685,
1911,
1324,
3304,
273,
13,
88,
4029,
8973,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
318,
7753,
7,
22179,
6978,
7,
35339,
62,
15908,
11,
27071,
1324,
3304,
273,
13,
88,
4029,
48774,
198,
220,
220,
220,
220,
220,
220,
220,
598,
3304,
273,
796,
1100,
7,
22179,
6978,
7,
35339,
62,
15908,
11,
27071,
1324,
3304,
273,
13,
88,
4029,
12340,
10903,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
5145,
13966,
1834,
259,
7203,
261,
62,
13138,
1600,
598,
3304,
273,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
5145,
13966,
1834,
259,
7203,
4,
41,
43,
62,
34,
3727,
2943,
8874,
62,
6173,
46023,
4,
1600,
598,
3304,
273,
8,
198,
220,
220,
220,
220,
220,
220,
220,
42721,
7,
22179,
6978,
7,
35339,
62,
15908,
11,
27071,
1324,
3304,
273,
13,
88,
4029,
48774,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
2980,
803,
262,
13877,
351,
39298,
709,
287,
262,
11055,
815,
2251,
257,
1281,
12,
9288,
2239,
13,
198,
220,
220,
220,
220,
220,
220,
220,
256,
13,
37390,
58,
43806,
721,
709,
60,
796,
39298,
709,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2429,
62,
33803,
7,
79,
11,
256,
11,
1332,
62,
35339,
8,
198,
220,
220,
220,
220,
220,
220,
220,
12233,
0,
7,
83,
13,
37390,
11,
39298,
709,
8,
198,
220,
220,
220,
220,
220,
220,
220,
598,
3304,
273,
796,
1100,
7,
22179,
6978,
7,
35339,
62,
15908,
11,
27071,
1324,
3304,
273,
13,
88,
4029,
12340,
10903,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
8833,
259,
7203,
261,
62,
13138,
1600,
598,
3304,
273,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
8833,
259,
7203,
4,
41,
43,
62,
34,
3727,
2943,
8874,
62,
6173,
46023,
4,
1600,
598,
3304,
273,
8,
198,
220,
220,
220,
220,
220,
220,
220,
42721,
7,
22179,
6978,
7,
35339,
62,
15908,
11,
27071,
1324,
3304,
273,
13,
88,
4029,
48774,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
16926,
46,
25,
3060,
17546,
5691,
5254,
618,
2034,
53,
2959,
273,
13,
20362,
6971,
340,
13,
628,
220,
220,
220,
220,
220,
220,
220,
279,
796,
2034,
53,
2959,
273,
7,
26,
4566,
62,
7753,
28,
22366,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
318,
28920,
7,
5235,
62,
33803,
7,
79,
11,
256,
11,
1332,
62,
35339,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
5145,
4468,
576,
7,
22179,
6978,
7,
35339,
62,
15908,
11,
27071,
1324,
3304,
273,
13,
88,
4029,
48774,
198,
220,
220,
220,
886,
198,
437,
198,
198,
26224,
7,
35339,
62,
15908,
26,
45115,
28,
7942,
8,
198
] | 2.093002 | 1,086 |
using Documenter
using MPIMeasurements
makedocs(
sitename = "MPIMeasurements",
authors = "Tobias Knopp et al.",
format = Documenter.HTML(prettyurls = false),
modules = [MPIMeasurements],
pages = [
"Home" => "index.md",
"Manual" => Any[
"Guide" => "man/guide.md",
"Devices" => "man/devices.md",
"Protocols" => "man/protocols.md",
"Sequences" => "man/sequences.md",
"Examples" => "man/examples.md",
],
"Library" => Any[
"Public" => "lib/public.md",
"Internals" => map(
s -> "lib/internals/$(s)",
sort(readdir(normpath(@__DIR__, "src/lib/internals")))
),
],
],
)
# deploydocs(repo = "github.com/MagneticParticleImaging/MPIMeasurements.jl.git",
# target = "build")
| [
3500,
16854,
263,
198,
3500,
4904,
3955,
68,
5015,
902,
198,
198,
76,
4335,
420,
82,
7,
198,
220,
220,
220,
1650,
12453,
796,
366,
7378,
3955,
68,
5015,
902,
1600,
198,
220,
220,
220,
7035,
796,
366,
51,
672,
4448,
509,
3919,
381,
2123,
435,
33283,
198,
220,
220,
220,
5794,
796,
16854,
263,
13,
28656,
7,
37784,
6371,
82,
796,
3991,
828,
198,
220,
220,
220,
13103,
796,
685,
7378,
3955,
68,
5015,
902,
4357,
198,
220,
220,
220,
5468,
796,
685,
198,
220,
220,
220,
220,
220,
220,
220,
366,
16060,
1,
5218,
366,
9630,
13,
9132,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
366,
5124,
723,
1,
5218,
4377,
58,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
47889,
1,
5218,
366,
805,
14,
41311,
13,
9132,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
13603,
1063,
1,
5218,
366,
805,
14,
42034,
13,
9132,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
19703,
4668,
82,
1,
5218,
366,
805,
14,
11235,
4668,
82,
13,
9132,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
44015,
3007,
1,
5218,
366,
805,
14,
3107,
3007,
13,
9132,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
27730,
1,
5218,
366,
805,
14,
1069,
12629,
13,
9132,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
16589,
198,
220,
220,
220,
220,
220,
220,
220,
366,
23377,
1,
5218,
4377,
58,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
15202,
1,
5218,
366,
8019,
14,
11377,
13,
9132,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
15865,
874,
1,
5218,
3975,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
264,
4613,
366,
8019,
14,
23124,
874,
32624,
7,
82,
42501,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3297,
7,
961,
15908,
7,
27237,
6978,
7,
31,
834,
34720,
834,
11,
366,
10677,
14,
8019,
14,
23124,
874,
1,
22305,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
10612,
198,
220,
220,
220,
220,
220,
220,
220,
16589,
198,
220,
220,
220,
16589,
198,
8,
198,
198,
2,
6061,
31628,
7,
260,
7501,
220,
220,
796,
366,
12567,
13,
785,
14,
13436,
9833,
7841,
1548,
3546,
3039,
14,
7378,
3955,
68,
5015,
902,
13,
20362,
13,
18300,
1600,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2496,
796,
366,
11249,
4943,
198
] | 1.930958 | 449 |
using GuessworkQuantumSideInfo
using SDPAFamily
using Test
using LinearAlgebra, GenericLinearAlgebra
# Fix a compatibility problem
LinearAlgebra.eigmin(A::Hermitian{Complex{BigFloat},Array{Complex{BigFloat},2}}) =
minimum(real.(eigvals(A)))
function testPOVM(Es; tol = 1e-25)
dB = size(first(Es), 1)
@test sum(Es) ≈ complex(1.0) * I(dB) atol = tol
for E in Es
@test E ≈ E' atol = tol
end
@test all(isposdef, Hermitian(E) + tol * I(dB) for E in Es)
end
T = BigFloat
default_sdp_solver(T) = SDPAFamily.Optimizer{T}(presolve = true)
@testset "BB84" begin
ρBs = GuessworkQuantumSideInfo.BB84_states(T)
p = ones(T, 4) / 4
output = guesswork(p, ρBs; solver = default_sdp_solver(T))
testPOVM(output.Es)
relaxed_output =
guesswork_upper_bound(p, ρBs; num_constraints = 4, make_solver = () -> default_sdp_solver(T))
true_val = (big(1) / big(4)) * (10 - sqrt(big(10)))
@test output.optval ≈ true_val rtol = 1e-25
@test output.optval ≈ relaxed_output.optval rtol = 1e-4
lb = guesswork_lower_bound(p, ρBs; solver = default_sdp_solver(T)).optval
@test lb <= output.optval + 1e-4
end
| [
3500,
37571,
1818,
24915,
388,
24819,
12360,
198,
3500,
9834,
4537,
24094,
198,
3500,
6208,
198,
3500,
44800,
2348,
29230,
11,
42044,
14993,
451,
2348,
29230,
198,
198,
2,
13268,
257,
17764,
1917,
198,
14993,
451,
2348,
29230,
13,
68,
328,
1084,
7,
32,
3712,
9360,
2781,
666,
90,
5377,
11141,
90,
12804,
43879,
5512,
19182,
90,
5377,
11141,
90,
12804,
43879,
5512,
17,
11709,
8,
796,
198,
220,
220,
220,
5288,
7,
5305,
12195,
68,
328,
12786,
7,
32,
22305,
628,
198,
8818,
1332,
47,
8874,
44,
7,
23041,
26,
284,
75,
796,
352,
68,
12,
1495,
8,
198,
220,
220,
220,
30221,
796,
2546,
7,
11085,
7,
23041,
828,
352,
8,
198,
220,
220,
220,
2488,
9288,
2160,
7,
23041,
8,
15139,
230,
3716,
7,
16,
13,
15,
8,
1635,
314,
7,
36077,
8,
379,
349,
796,
284,
75,
628,
220,
220,
220,
329,
412,
287,
8678,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
412,
15139,
230,
412,
6,
379,
349,
796,
284,
75,
198,
220,
220,
220,
886,
198,
220,
220,
220,
2488,
9288,
477,
7,
271,
1930,
4299,
11,
2332,
2781,
666,
7,
36,
8,
1343,
284,
75,
1635,
314,
7,
36077,
8,
329,
412,
287,
8678,
8,
198,
437,
198,
198,
51,
796,
4403,
43879,
198,
12286,
62,
21282,
79,
62,
82,
14375,
7,
51,
8,
796,
9834,
4537,
24094,
13,
27871,
320,
7509,
90,
51,
92,
7,
18302,
6442,
796,
2081,
8,
198,
198,
31,
9288,
2617,
366,
15199,
5705,
1,
2221,
198,
220,
220,
220,
18074,
223,
37000,
796,
37571,
1818,
24915,
388,
24819,
12360,
13,
15199,
5705,
62,
27219,
7,
51,
8,
628,
220,
220,
220,
279,
796,
3392,
7,
51,
11,
604,
8,
1220,
604,
198,
220,
220,
220,
5072,
796,
4724,
1818,
7,
79,
11,
18074,
223,
37000,
26,
1540,
332,
796,
4277,
62,
21282,
79,
62,
82,
14375,
7,
51,
4008,
198,
220,
220,
220,
1332,
47,
8874,
44,
7,
22915,
13,
23041,
8,
198,
220,
220,
220,
18397,
62,
22915,
796,
198,
220,
220,
220,
220,
220,
220,
220,
4724,
1818,
62,
45828,
62,
7784,
7,
79,
11,
18074,
223,
37000,
26,
997,
62,
1102,
2536,
6003,
796,
604,
11,
787,
62,
82,
14375,
796,
7499,
4613,
4277,
62,
21282,
79,
62,
82,
14375,
7,
51,
4008,
628,
220,
220,
220,
2081,
62,
2100,
796,
357,
14261,
7,
16,
8,
1220,
1263,
7,
19,
4008,
1635,
357,
940,
532,
19862,
17034,
7,
14261,
7,
940,
22305,
198,
220,
220,
220,
2488,
9288,
5072,
13,
8738,
2100,
15139,
230,
2081,
62,
2100,
374,
83,
349,
796,
352,
68,
12,
1495,
628,
220,
220,
220,
2488,
9288,
5072,
13,
8738,
2100,
15139,
230,
18397,
62,
22915,
13,
8738,
2100,
374,
83,
349,
796,
352,
68,
12,
19,
628,
220,
220,
220,
18360,
796,
4724,
1818,
62,
21037,
62,
7784,
7,
79,
11,
18074,
223,
37000,
26,
1540,
332,
796,
4277,
62,
21282,
79,
62,
82,
14375,
7,
51,
29720,
8738,
2100,
198,
220,
220,
220,
2488,
9288,
18360,
19841,
5072,
13,
8738,
2100,
1343,
352,
68,
12,
19,
198,
437,
198
] | 2.256809 | 514 |
"""
get_observable_timers() :: Nothing
Test performance of current observable implementation by Fourier transforming test correlations
on a few 2D and 3D lattices with 50 x 50 x 0 (2D) or 50 x 50 x 50 (3D) momenta.
"""
function get_observable_timers() :: Nothing
# init test dummys
l1 = get_lattice("square", 6, verbose = false); r1 = get_reduced_lattice("heisenberg", [[0.0]], l1, verbose = false)
l2 = get_lattice("cubic", 6, verbose = false); r2 = get_reduced_lattice("heisenberg", [[0.0]], l2, verbose = false)
l3 = get_lattice("kagome", 6, verbose = false); r3 = get_reduced_lattice("heisenberg", [[0.0]], l3, verbose = false)
l4 = get_lattice("hyperkagome", 6, verbose = false); r4 = get_reduced_lattice("heisenberg", [[0.0]], l4, verbose = false)
χ1 = Float64[exp(-norm(r1.sites[i].vec)) for i in eachindex(r1.sites)]
χ2 = Float64[exp(-norm(r2.sites[i].vec)) for i in eachindex(r2.sites)]
χ3 = Float64[exp(-norm(r3.sites[i].vec)) for i in eachindex(r3.sites)]
χ4 = Float64[exp(-norm(r4.sites[i].vec)) for i in eachindex(r4.sites)]
k_2D = get_momenta((0.0, 1.0 * pi), (0.0, 1.0 * pi), (0.0, 0.0), (50, 50, 0))
k_3D = get_momenta((0.0, 1.0 * pi), (0.0, 1.0 * pi), (0.0, 1.0 * pi), (50, 50, 50))
# init timer
to = TimerOutput()
@timeit to "=> Fourier transform" begin
for rep in 1 : 10
@timeit to "-> square" compute_structure_factor(χ1, k_2D, l1, r1)
@timeit to "-> cubic" compute_structure_factor(χ2, k_3D, l2, r2)
@timeit to "-> kagome" compute_structure_factor(χ3, k_2D, l3, r3)
@timeit to "-> hyperkagome" compute_structure_factor(χ4, k_3D, l4, r4)
end
end
show(to)
return nothing
end | [
37811,
198,
220,
220,
220,
651,
62,
672,
3168,
540,
62,
16514,
364,
3419,
7904,
10528,
220,
198,
198,
14402,
2854,
286,
1459,
42550,
7822,
416,
34296,
5277,
25449,
1332,
35811,
220,
198,
261,
257,
1178,
362,
35,
290,
513,
35,
47240,
1063,
351,
2026,
2124,
2026,
2124,
657,
357,
17,
35,
8,
393,
2026,
2124,
2026,
2124,
2026,
357,
18,
35,
8,
2589,
64,
13,
198,
37811,
198,
8818,
651,
62,
672,
3168,
540,
62,
16514,
364,
3419,
7904,
10528,
628,
220,
220,
220,
1303,
2315,
1332,
288,
13929,
893,
198,
220,
220,
220,
300,
16,
220,
220,
796,
651,
62,
75,
1078,
501,
7203,
23415,
1600,
220,
220,
220,
220,
220,
718,
11,
15942,
577,
796,
3991,
1776,
374,
16,
796,
651,
62,
445,
19513,
62,
75,
1078,
501,
7203,
258,
13254,
3900,
1600,
16410,
15,
13,
15,
60,
4357,
300,
16,
11,
15942,
577,
796,
3991,
8,
198,
220,
220,
220,
300,
17,
220,
220,
796,
651,
62,
75,
1078,
501,
7203,
66,
549,
291,
1600,
220,
220,
220,
220,
220,
220,
718,
11,
15942,
577,
796,
3991,
1776,
374,
17,
796,
651,
62,
445,
19513,
62,
75,
1078,
501,
7203,
258,
13254,
3900,
1600,
16410,
15,
13,
15,
60,
4357,
300,
17,
11,
15942,
577,
796,
3991,
8,
198,
220,
220,
220,
300,
18,
220,
220,
796,
651,
62,
75,
1078,
501,
7203,
74,
363,
462,
1600,
220,
220,
220,
220,
220,
718,
11,
15942,
577,
796,
3991,
1776,
374,
18,
796,
651,
62,
445,
19513,
62,
75,
1078,
501,
7203,
258,
13254,
3900,
1600,
16410,
15,
13,
15,
60,
4357,
300,
18,
11,
15942,
577,
796,
3991,
8,
198,
220,
220,
220,
300,
19,
220,
220,
796,
651,
62,
75,
1078,
501,
7203,
49229,
74,
363,
462,
1600,
718,
11,
15942,
577,
796,
3991,
1776,
374,
19,
796,
651,
62,
445,
19513,
62,
75,
1078,
501,
7203,
258,
13254,
3900,
1600,
16410,
15,
13,
15,
60,
4357,
300,
19,
11,
15942,
577,
796,
3991,
8,
198,
220,
220,
220,
18074,
229,
16,
220,
220,
796,
48436,
2414,
58,
11201,
32590,
27237,
7,
81,
16,
13,
49315,
58,
72,
4083,
35138,
4008,
329,
1312,
287,
1123,
9630,
7,
81,
16,
13,
49315,
15437,
198,
220,
220,
220,
18074,
229,
17,
220,
220,
796,
48436,
2414,
58,
11201,
32590,
27237,
7,
81,
17,
13,
49315,
58,
72,
4083,
35138,
4008,
329,
1312,
287,
1123,
9630,
7,
81,
17,
13,
49315,
15437,
198,
220,
220,
220,
18074,
229,
18,
220,
220,
796,
48436,
2414,
58,
11201,
32590,
27237,
7,
81,
18,
13,
49315,
58,
72,
4083,
35138,
4008,
329,
1312,
287,
1123,
9630,
7,
81,
18,
13,
49315,
15437,
198,
220,
220,
220,
18074,
229,
19,
220,
220,
796,
48436,
2414,
58,
11201,
32590,
27237,
7,
81,
19,
13,
49315,
58,
72,
4083,
35138,
4008,
329,
1312,
287,
1123,
9630,
7,
81,
19,
13,
49315,
15437,
198,
220,
220,
220,
479,
62,
17,
35,
796,
651,
62,
32542,
29188,
19510,
15,
13,
15,
11,
352,
13,
15,
1635,
31028,
828,
357,
15,
13,
15,
11,
352,
13,
15,
1635,
31028,
828,
357,
15,
13,
15,
11,
657,
13,
15,
828,
357,
1120,
11,
2026,
11,
657,
4008,
198,
220,
220,
220,
479,
62,
18,
35,
796,
651,
62,
32542,
29188,
19510,
15,
13,
15,
11,
352,
13,
15,
1635,
31028,
828,
357,
15,
13,
15,
11,
352,
13,
15,
1635,
31028,
828,
357,
15,
13,
15,
11,
352,
13,
15,
1635,
31028,
828,
357,
1120,
11,
2026,
11,
2026,
4008,
628,
220,
220,
220,
1303,
2315,
19781,
198,
220,
220,
220,
284,
796,
5045,
263,
26410,
3419,
628,
220,
220,
220,
2488,
2435,
270,
284,
366,
14804,
34296,
5277,
6121,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
329,
1128,
287,
352,
1058,
838,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
2435,
270,
284,
366,
3784,
6616,
1,
220,
220,
220,
220,
220,
24061,
62,
301,
5620,
62,
31412,
7,
139,
229,
16,
11,
479,
62,
17,
35,
11,
300,
16,
11,
374,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
2435,
270,
284,
366,
3784,
27216,
1,
220,
220,
220,
220,
220,
220,
24061,
62,
301,
5620,
62,
31412,
7,
139,
229,
17,
11,
479,
62,
18,
35,
11,
300,
17,
11,
374,
17,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
2435,
270,
284,
366,
3784,
479,
363,
462,
1,
220,
220,
220,
220,
220,
24061,
62,
301,
5620,
62,
31412,
7,
139,
229,
18,
11,
479,
62,
17,
35,
11,
300,
18,
11,
374,
18,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
2435,
270,
284,
366,
3784,
8718,
74,
363,
462,
1,
24061,
62,
301,
5620,
62,
31412,
7,
139,
229,
19,
11,
479,
62,
18,
35,
11,
300,
19,
11,
374,
19,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
220,
198,
220,
220,
220,
886,
628,
220,
220,
220,
905,
7,
1462,
8,
628,
220,
220,
220,
1441,
2147,
220,
198,
437
] | 2.104829 | 849 |
# FUNCTION recover_primal
"""Computes the Ridge regressor
INPUT
ℓ - LossFunction to use
Y - Vector of observed responses
Z - Matrix of observed features
γ - Regularization parameter
OUTPUT
w - Optimal regressor"""
function recover_primal(ℓ::Regression, Y, Z, γ)
CM = Matrix(I, size(Z,2), size(Z,2))/γ + Z'*Z # The capacitance matrix
α = -Y + Z*(CM\(Z'*Y)) # Matrix Inversion Lemma
return -γ*Z'*α # Regressor
end
using LIBLINEAR
function recover_primal(ℓ::Classification, Y, Z, γ)
solverNumber = LibLinearSolver(ℓ)
if isa(ℓ, SubsetSelection.Classification)
model = LIBLINEAR.linear_train(Y, Z'; verbose=false, C=γ, solver_type=Cint(solverNumber))
return Y[1]*model.w
# else
# model = linear_train(Y, Z'; verbose=false, C=γ, solver_type=Cint(solverNumber), eps = ℓ.ε)
# return model.w
end
end
function LibLinearSolver(ℓ::L1SVR)
return 13
end
function LibLinearSolver(ℓ::L2SVR)
return 12
end
function LibLinearSolver(ℓ::LogReg)
return 7
end
function LibLinearSolver(ℓ::L1SVM)
return 3
end
function LibLinearSolver(ℓ::L2SVM)
return 2
end
| [
2,
29397,
4177,
2849,
8551,
62,
1050,
4402,
198,
37811,
7293,
1769,
262,
20614,
842,
44292,
198,
1268,
30076,
198,
220,
2343,
226,
241,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
532,
22014,
22203,
284,
779,
198,
220,
575,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
532,
20650,
286,
6515,
9109,
198,
220,
1168,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
532,
24936,
286,
6515,
3033,
198,
220,
7377,
111,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
532,
23603,
1634,
11507,
198,
2606,
7250,
3843,
198,
220,
266,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
532,
13123,
4402,
842,
44292,
37811,
198,
8818,
8551,
62,
1050,
4402,
7,
158,
226,
241,
3712,
8081,
2234,
11,
575,
11,
1168,
11,
7377,
111,
8,
198,
220,
16477,
796,
24936,
7,
40,
11,
2546,
7,
57,
11,
17,
828,
2546,
7,
57,
11,
17,
4008,
14,
42063,
1343,
1168,
6,
9,
57,
220,
220,
220,
220,
220,
1303,
383,
18457,
42942,
17593,
198,
220,
26367,
796,
532,
56,
1343,
1168,
9,
7,
24187,
59,
7,
57,
6,
9,
56,
4008,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
24936,
554,
9641,
20607,
2611,
198,
220,
1441,
532,
42063,
9,
57,
6,
9,
17394,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
3310,
44292,
198,
437,
198,
198,
3500,
24653,
9148,
8881,
1503,
198,
198,
8818,
8551,
62,
1050,
4402,
7,
158,
226,
241,
3712,
9487,
2649,
11,
575,
11,
1168,
11,
7377,
111,
8,
198,
220,
1540,
332,
15057,
796,
7980,
14993,
451,
50,
14375,
7,
158,
226,
241,
8,
198,
220,
611,
318,
64,
7,
158,
226,
241,
11,
3834,
2617,
4653,
1564,
13,
9487,
2649,
8,
198,
220,
220,
220,
2746,
796,
24653,
9148,
8881,
1503,
13,
29127,
62,
27432,
7,
56,
11,
1168,
17020,
15942,
577,
28,
9562,
11,
327,
28,
42063,
11,
1540,
332,
62,
4906,
28,
34,
600,
7,
82,
14375,
15057,
4008,
198,
220,
220,
220,
1441,
575,
58,
16,
60,
9,
19849,
13,
86,
198,
220,
1303,
2073,
198,
220,
1303,
220,
220,
2746,
796,
14174,
62,
27432,
7,
56,
11,
1168,
17020,
15942,
577,
28,
9562,
11,
327,
28,
42063,
11,
1540,
332,
62,
4906,
28,
34,
600,
7,
82,
14375,
15057,
828,
304,
862,
796,
2343,
226,
241,
13,
30950,
8,
198,
220,
1303,
220,
220,
1441,
2746,
13,
86,
198,
220,
886,
198,
437,
628,
198,
8818,
7980,
14993,
451,
50,
14375,
7,
158,
226,
241,
3712,
43,
16,
50,
13024,
8,
198,
220,
1441,
1511,
198,
437,
198,
8818,
7980,
14993,
451,
50,
14375,
7,
158,
226,
241,
3712,
43,
17,
50,
13024,
8,
198,
220,
1441,
1105,
198,
437,
198,
198,
8818,
7980,
14993,
451,
50,
14375,
7,
158,
226,
241,
3712,
11187,
8081,
8,
198,
220,
1441,
767,
198,
437,
198,
8818,
7980,
14993,
451,
50,
14375,
7,
158,
226,
241,
3712,
43,
16,
50,
15996,
8,
198,
220,
1441,
513,
198,
437,
198,
8818,
7980,
14993,
451,
50,
14375,
7,
158,
226,
241,
3712,
43,
17,
50,
15996,
8,
198,
220,
1441,
362,
198,
437,
198
] | 2.182156 | 538 |
struct Euler <: Equation end
@declare_dofs Euler [:rhou, :rhov, :rho, :e]
struct GaussianWaveeuler <: Scenario end
struct Sod_shock_tube <: Scenario end
function is_periodic_boundary(equation::Euler, scenario::GaussianWaveeuler)
# GaussianWave scenario with periodic boundary conditions
true
end
function is_periodic_boundary(equation::Euler, scenario::Sod_shock_tube)
# sod_shock_tube scenario doesn't require periodic boundary conditions
false
end
# for Sod_shock_tube Scenario
function evaluate_boundary(eq::Euler, scenario::Sod_shock_tube, face, normalidx, dofsface, dofsfaceneigh)
# dofsface and dofsfaceneigh have shape (num_2d_quadpoints, dofs)
# you need to set dofsfaceneigh
dofsfaceneigh .= dofsface
if normalidx == 1
dofsfaceneigh[:, 1] = -dofsface[:, 1]
else
dofsfaceneigh[:, 2] = -dofsface[:, 2]
end
end
function evaluate_energy(eq::Euler, rhou, rhov, rho, p)
# value of gamma is 1.4, hence gamma-1 = 0.4
return ( (p/0.4) + (1/(2*rho)) * (rhou^2 + rhov^2) )
end
function evaluate_pressure(eq::Euler, rhou, rhov, rho, e)
# value of gamma is 1.4, hence gamma-1 = 0.4
return ( (0.4) * ( e - (1/(2*rho)) * (rhou^2 + rhov^2) ))
end
function get_initial_values(eq::Euler, scenario::GaussianWaveeuler, global_position; t=0.0)
x, y = global_position
if is_analytical_solution(eq, scenario)
println("No analytical solution implemented")
else
p = exp(-100 * (x - 0.5)^2 - 100 *(y - 0.5)^2) + 1
[0, 0, 1.0, evaluate_energy(eq, 0, 0, 1.0, p)]
end
end
function get_initial_values(eq::Euler, scenario::Sod_shock_tube, global_position; t=0.0)
x, y = global_position
if is_analytical_solution(eq, scenario)
println("No analytical solution implemented")
else
[ 0, 0, if x<=0.5 0.125 else 1.0 end, if x<= 0.5 evaluate_energy(eq, 0, 0, 0.125, 0.1) else evaluate_energy(eq, 0, 0, 1.0, 1.0) end ]
end
end
function is_analytical_solution(equation::Euler, scenario::GaussianWaveeuler)
false
end
function is_analytical_solution(equation::Euler, scenario::Sod_shock_tube)
false
end
function evaluate_flux(eq::Euler, celldofs, cellflux)
# size(cellflux)=(2*order*order, ndof)=(2,5)
# size(celldofs)=(order*order, ndof)=(1,5)
order_sq, ndof = size(celldofs)
for j=1:order_sq
# explicit names of dofs for clarity
rhou = celldofs[j, 1]
rhov = celldofs[j, 2]
density = celldofs[j, 3]
e = celldofs[j, 4]
p=evaluate_pressure(eq,rhou,rhov,density,e)
# here flux is computed elementwise
cellflux[j,1]= (1/density)*rhou^2 + p
cellflux[j + order_sq, 1] = (1/density)*rhou*rhov
cellflux[j,2] = (1/density)*rhou*rhov
cellflux[j + order_sq, 2] = (1/density)*rhov^2 + p
cellflux[j,3] = rhou
cellflux[j + order_sq, 3] = rhov
cellflux[j,4] = (rhou/density)*(e+p)
cellflux[j + order_sq, 4] = (rhov/density)*(e+p)
end
end
function max_eigenval(eq::Euler, celldata, normalidx)
# v_n + c, where c = sqrt(gamma*p /rho)
maxeigenval = 0.
#@info size(celldata)
for i in 1:size(celldata)[1]
rhou, rhov, density, e = celldata[i, :]
pressure = evaluate_pressure(eq,rhou,rhov,density,e)
#@info i
#@info pressure
#@info density
#@info e
#@info rhou
#@info rhov
c = sqrt(1.4*pressure/density)
if normalidx == 1
vn = rhou / density
else
vn = rhov / density
end
maxeigenval_new = vn + c
if maxeigenval_new > maxeigenval
maxeigenval = maxeigenval_new
end
end
maxeigenval
end
| [
7249,
412,
18173,
1279,
25,
7889,
341,
886,
198,
31,
32446,
533,
62,
67,
1659,
82,
412,
18173,
685,
25,
81,
15710,
11,
1058,
17179,
709,
11,
1058,
81,
8873,
11,
1058,
68,
60,
198,
198,
7249,
12822,
31562,
39709,
68,
18173,
1279,
25,
1446,
39055,
886,
198,
7249,
43829,
62,
39563,
62,
29302,
1279,
25,
1446,
39055,
886,
628,
198,
8818,
318,
62,
41007,
291,
62,
7784,
560,
7,
4853,
341,
3712,
36,
18173,
11,
8883,
3712,
35389,
31562,
39709,
68,
18173,
8,
198,
220,
220,
220,
1303,
12822,
31562,
39709,
8883,
351,
27458,
18645,
3403,
198,
220,
220,
220,
2081,
198,
437,
198,
198,
8818,
318,
62,
41007,
291,
62,
7784,
560,
7,
4853,
341,
3712,
36,
18173,
11,
8883,
3712,
50,
375,
62,
39563,
62,
29302,
8,
198,
220,
220,
220,
1303,
32581,
62,
39563,
62,
29302,
8883,
1595,
470,
2421,
27458,
18645,
3403,
198,
220,
220,
220,
3991,
198,
437,
198,
198,
2,
329,
43829,
62,
39563,
62,
29302,
1446,
39055,
198,
8818,
13446,
62,
7784,
560,
7,
27363,
3712,
36,
18173,
11,
8883,
3712,
50,
375,
62,
39563,
62,
29302,
11,
1986,
11,
3487,
312,
87,
11,
466,
9501,
2550,
11,
466,
9501,
38942,
1734,
394,
8,
198,
2,
466,
9501,
2550,
290,
466,
9501,
38942,
1734,
394,
423,
5485,
357,
22510,
62,
17,
67,
62,
47003,
13033,
11,
466,
9501,
8,
198,
2,
345,
761,
284,
900,
466,
9501,
38942,
1734,
394,
198,
220,
220,
220,
466,
9501,
38942,
1734,
394,
764,
28,
466,
9501,
2550,
198,
220,
220,
220,
611,
3487,
312,
87,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
466,
9501,
38942,
1734,
394,
58,
45299,
352,
60,
796,
532,
67,
1659,
82,
2550,
58,
45299,
352,
60,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
466,
9501,
38942,
1734,
394,
58,
45299,
362,
60,
796,
532,
67,
1659,
82,
2550,
58,
45299,
362,
60,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
13446,
62,
22554,
7,
27363,
3712,
36,
18173,
11,
9529,
280,
11,
9529,
709,
11,
374,
8873,
11,
279,
8,
198,
220,
220,
220,
1303,
1988,
286,
34236,
318,
352,
13,
19,
11,
12891,
34236,
12,
16,
796,
657,
13,
19,
198,
220,
220,
220,
1441,
357,
357,
79,
14,
15,
13,
19,
8,
220,
1343,
220,
357,
16,
29006,
17,
9,
81,
8873,
4008,
1635,
357,
81,
15710,
61,
17,
1343,
9529,
709,
61,
17,
8,
220,
1267,
198,
437,
198,
198,
8818,
13446,
62,
36151,
7,
27363,
3712,
36,
18173,
11,
9529,
280,
11,
9529,
709,
11,
374,
8873,
11,
304,
8,
198,
220,
220,
220,
1303,
1988,
286,
34236,
318,
352,
13,
19,
11,
12891,
34236,
12,
16,
796,
657,
13,
19,
198,
220,
220,
220,
1441,
357,
357,
15,
13,
19,
8,
1635,
357,
304,
532,
357,
16,
29006,
17,
9,
81,
8873,
4008,
1635,
357,
81,
15710,
61,
17,
1343,
9529,
709,
61,
17,
8,
15306,
198,
437,
198,
198,
8818,
651,
62,
36733,
62,
27160,
7,
27363,
3712,
36,
18173,
11,
8883,
3712,
35389,
31562,
39709,
68,
18173,
11,
3298,
62,
9150,
26,
256,
28,
15,
13,
15,
8,
198,
220,
220,
220,
2124,
11,
331,
796,
3298,
62,
9150,
198,
220,
220,
220,
611,
318,
62,
38200,
22869,
62,
82,
2122,
7,
27363,
11,
8883,
8,
198,
220,
220,
220,
220,
220,
220,
220,
44872,
7203,
2949,
30063,
4610,
9177,
4943,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
279,
796,
1033,
32590,
3064,
1635,
357,
87,
532,
657,
13,
20,
8,
61,
17,
532,
1802,
1635,
7,
88,
532,
657,
13,
20,
8,
61,
17,
8,
1343,
352,
198,
220,
220,
220,
220,
220,
220,
220,
685,
15,
11,
657,
11,
352,
13,
15,
11,
13446,
62,
22554,
7,
27363,
11,
657,
11,
657,
11,
352,
13,
15,
11,
279,
15437,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
651,
62,
36733,
62,
27160,
7,
27363,
3712,
36,
18173,
11,
8883,
3712,
50,
375,
62,
39563,
62,
29302,
11,
3298,
62,
9150,
26,
256,
28,
15,
13,
15,
8,
198,
220,
220,
220,
2124,
11,
331,
796,
3298,
62,
9150,
198,
220,
220,
220,
611,
318,
62,
38200,
22869,
62,
82,
2122,
7,
27363,
11,
8883,
8,
198,
220,
220,
220,
220,
220,
220,
220,
44872,
7203,
2949,
30063,
4610,
9177,
4943,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
685,
657,
11,
657,
11,
611,
2124,
27,
28,
15,
13,
20,
657,
13,
11623,
2073,
352,
13,
15,
886,
11,
611,
2124,
27,
28,
657,
13,
20,
13446,
62,
22554,
7,
27363,
11,
657,
11,
657,
11,
657,
13,
11623,
11,
657,
13,
16,
8,
2073,
13446,
62,
22554,
7,
27363,
11,
657,
11,
657,
11,
352,
13,
15,
11,
352,
13,
15,
8,
886,
2361,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
318,
62,
38200,
22869,
62,
82,
2122,
7,
4853,
341,
3712,
36,
18173,
11,
8883,
3712,
35389,
31562,
39709,
68,
18173,
8,
198,
220,
220,
220,
3991,
198,
437,
198,
198,
8818,
318,
62,
38200,
22869,
62,
82,
2122,
7,
4853,
341,
3712,
36,
18173,
11,
8883,
3712,
50,
375,
62,
39563,
62,
29302,
8,
198,
220,
220,
220,
3991,
198,
437,
198,
198,
8818,
13446,
62,
69,
22564,
7,
27363,
3712,
36,
18173,
11,
2685,
67,
1659,
82,
11,
2685,
69,
22564,
8,
198,
220,
220,
220,
1303,
2546,
7,
3846,
69,
22564,
35793,
17,
9,
2875,
9,
2875,
11,
299,
67,
1659,
35793,
17,
11,
20,
8,
198,
220,
220,
220,
1303,
2546,
7,
3846,
67,
1659,
82,
35793,
2875,
9,
2875,
11,
299,
67,
1659,
35793,
16,
11,
20,
8,
628,
220,
220,
220,
1502,
62,
31166,
11,
299,
67,
1659,
796,
2546,
7,
3846,
67,
1659,
82,
8,
198,
220,
220,
220,
329,
474,
28,
16,
25,
2875,
62,
31166,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
7952,
3891,
286,
466,
9501,
329,
16287,
198,
220,
220,
220,
220,
220,
220,
220,
9529,
280,
796,
2685,
67,
1659,
82,
58,
73,
11,
352,
60,
198,
220,
220,
220,
220,
220,
220,
220,
9529,
709,
796,
2685,
67,
1659,
82,
58,
73,
11,
362,
60,
198,
220,
220,
220,
220,
220,
220,
220,
12109,
796,
2685,
67,
1659,
82,
58,
73,
11,
513,
60,
198,
220,
220,
220,
220,
220,
220,
220,
304,
796,
2685,
67,
1659,
82,
58,
73,
11,
604,
60,
628,
220,
220,
220,
220,
220,
220,
220,
279,
28,
49786,
62,
36151,
7,
27363,
11,
81,
15710,
11,
17179,
709,
11,
43337,
11,
68,
8,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
994,
28462,
318,
29231,
5002,
3083,
198,
220,
220,
220,
220,
220,
220,
220,
2685,
69,
22564,
58,
73,
11,
16,
22241,
357,
16,
14,
43337,
27493,
81,
15710,
61,
17,
1343,
279,
198,
220,
220,
220,
220,
220,
220,
220,
2685,
69,
22564,
58,
73,
1343,
1502,
62,
31166,
11,
352,
60,
796,
357,
16,
14,
43337,
27493,
81,
15710,
9,
17179,
709,
628,
220,
220,
220,
220,
220,
220,
220,
2685,
69,
22564,
58,
73,
11,
17,
60,
796,
357,
16,
14,
43337,
27493,
81,
15710,
9,
17179,
709,
198,
220,
220,
220,
220,
220,
220,
220,
2685,
69,
22564,
58,
73,
1343,
1502,
62,
31166,
11,
362,
60,
796,
357,
16,
14,
43337,
27493,
17179,
709,
61,
17,
1343,
279,
628,
220,
220,
220,
220,
220,
220,
220,
2685,
69,
22564,
58,
73,
11,
18,
60,
796,
9529,
280,
198,
220,
220,
220,
220,
220,
220,
220,
2685,
69,
22564,
58,
73,
1343,
1502,
62,
31166,
11,
513,
60,
796,
9529,
709,
628,
220,
220,
220,
220,
220,
220,
220,
2685,
69,
22564,
58,
73,
11,
19,
60,
796,
357,
81,
15710,
14,
43337,
27493,
7,
68,
10,
79,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2685,
69,
22564,
58,
73,
1343,
1502,
62,
31166,
11,
604,
60,
796,
357,
17179,
709,
14,
43337,
27493,
7,
68,
10,
79,
8,
628,
220,
220,
220,
886,
198,
198,
437,
198,
198,
8818,
3509,
62,
68,
9324,
2100,
7,
27363,
3712,
36,
18173,
11,
2685,
7890,
11,
3487,
312,
87,
8,
198,
220,
220,
220,
1303,
410,
62,
77,
1343,
269,
11,
810,
269,
796,
19862,
17034,
7,
28483,
2611,
9,
79,
1220,
81,
8873,
8,
198,
220,
220,
220,
3509,
68,
9324,
2100,
796,
657,
13,
198,
220,
220,
220,
1303,
31,
10951,
2546,
7,
3846,
7890,
8,
198,
220,
220,
220,
329,
1312,
287,
352,
25,
7857,
7,
3846,
7890,
38381,
16,
60,
198,
220,
220,
220,
220,
220,
220,
220,
9529,
280,
11,
9529,
709,
11,
12109,
11,
304,
796,
2685,
7890,
58,
72,
11,
1058,
60,
198,
220,
220,
220,
220,
220,
220,
220,
3833,
796,
13446,
62,
36151,
7,
27363,
11,
81,
15710,
11,
17179,
709,
11,
43337,
11,
68,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
31,
10951,
1312,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
31,
10951,
3833,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
31,
10951,
12109,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
31,
10951,
304,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
31,
10951,
9529,
280,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
31,
10951,
9529,
709,
198,
220,
220,
220,
220,
220,
220,
220,
269,
796,
19862,
17034,
7,
16,
13,
19,
9,
36151,
14,
43337,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
3487,
312,
87,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
410,
77,
796,
9529,
280,
1220,
12109,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
410,
77,
796,
9529,
709,
1220,
12109,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
3509,
68,
9324,
2100,
62,
3605,
796,
410,
77,
1343,
269,
198,
220,
220,
220,
220,
220,
220,
220,
611,
3509,
68,
9324,
2100,
62,
3605,
1875,
3509,
68,
9324,
2100,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3509,
68,
9324,
2100,
796,
3509,
68,
9324,
2100,
62,
3605,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
3509,
68,
9324,
2100,
198,
437,
198
] | 2.146074 | 1,732 |
# file containing methods for requesting forecast simulations from GEOGloWS
"""
forecast_stats(parameters::AbstractDict{Symbol,<:Any})
returns statistics calculated from 51 forecast ensemble members.
"""
function forecast_stats(parameters::AbstractDict{Symbol,<:Any})
endpoint = "ForecastStats"
r = _make_request(baseurl, endpoint, parameters)
_read_response(r, parameters[:return_format], with_dates = true)
end
"""
forecast_stats(reach_id::Int; return_format::Symbol = :csv)
returns statistics calculated from 51 forecast ensemble members.
"""
function forecast_stats(reach_id::Int; return_format::Symbol = :csv)
parameters = Dict(:reach_id => reach_id, :return_format => return_format)
forecast_stats(parameters)
end
"""
forecast_stats(reach_id::Int,date::AbstractString; return_format::Symbol = :csv)
returns statistics calculated from 51 forecast ensemble members.
"""
function forecast_stats(
reach_id::Int,
date::AbstractString;
return_format::Symbol = :csv,
)
parameters = Dict(
:reach_id => reach_id,
:date => date,
:return_format => return_format,
)
forecast_stats(parameters)
end
"""
forecast_stats(reach_id::Int,date::TimeType; return_format::Symbol = :csv)
returns statistics calculated from 51 forecast ensemble members.
"""
function forecast_stats(
reach_id::Int,
date::TimeType;
return_format::Symbol = :csv,
)
datestr = try
Dates.format(date, "YYYY-m-d")
catch
Dates.format(date, "yyyymmdd")
end
parameters = Dict(
:reach_id => reach_id,
:date => datestr,
:return_format => return_format,
)
forecast_stats(parameters)
end
"""
forecast_stats(lat::Real, lon::Real; return_format::Symbol = :csv)
returns statistics calculated from 51 forecast ensemble members.
"""
function forecast_stats(lat::Real, lon::Real; return_format::Symbol = :csv)
parameters = Dict(:lat => lat, :lon => lon, :return_format => return_format)
forecast_stats(parameters)
end
"""
forecast_stats(lat::Real, lon::Real, date::AbstractString; return_format::Symbol = :csv)
returns statistics calculated from 51 forecast ensemble members.
"""
function forecast_stats(
lat::Real,
lon::Real,
date::AbstractString;
return_format::Symbol = :csv,
)
if occursin("-", date)
date = replace(date, "-" => "")
end
parameters = Dict(
:lat => lat,
:lon => lon,
:date => date,
:return_format => return_format,
)
forecast_stats(parameters)
end
"""
forecast_stats(lat::Real, lon::Real, date::TimeType; return_format::Symbol = :csv)
returns statistics calculated from 51 forecast ensemble members.
"""
function forecast_stats(
lat::Real,
lon::Real,
date::TimeType;
return_format::Symbol = :csv,
)
datestr = Dates.format(date, "yyyymmdd")
parameters = Dict(
:lat => lat,
:lon => lon,
:date => datestr,
:return_format => return_format,
)
forecast_stats(parameters)
end
"""
forecast_ensembles(parameters::AbstractDict{Symbol,<:Any})
returns a timeseries for each of the 51 normal forecast ensemble members and the 52nd higher resolution forecast
"""
function forecast_ensembles(parameters::AbstractDict{Symbol,<:Any})
endpoint = "ForecastEnsembles"
r = _make_request(baseurl, endpoint, parameters)
_read_response(r, parameters[:return_format], with_dates = true)
end
"""
forecast_ensembles(reach_id::Int; return_format::Symbol = :csv)
returns a timeseries for each of the 51 normal forecast ensemble members and the 52nd higher resolution forecast
"""
function forecast_ensembles(reach_id::Int; return_format::Symbol = :csv)
parameters = Dict(:reach_id => reach_id, :return_format => return_format)
forecast_ensembles(parameters)
end
"""
forecast_ensembles(reach_id::Int, date::AbstractString; return_format::Symbol = :csv)
returns a timeseries for each of the 51 normal forecast ensemble members and the 52nd higher resolution forecast
"""
function forecast_ensembles(
reach_id::Int,
date::AbstractString;
return_format::Symbol = :csv,
)
if occursin("-", date)
date = replace(date, "-" => "")
end
parameters = Dict(
:reach_id => reach_id,
:date => date,
:return_format => return_format,
)
forecast_ensembles(parameters)
end
"""
forecast_ensembles(reach_id::Int, date::TimeType; return_format::Symbol = :csv)
returns a timeseries for each of the 51 normal forecast ensemble members and the 52nd higher resolution forecast
"""
function forecast_ensembles(
reach_id::Int,
date::TimeType;
return_format::Symbol = :csv,
)
datestr = Dates.format(date, "yyyymmdd")
parameters = Dict(
:reach_id => reach_id,
:date => datestr,
:return_format => return_format,
)
forecast_ensembles(parameters)
end
"""
forecast_ensembles(lat::Real, lon::Real; return_format::Symbol = :csv)
returns a timeseries for each of the 51 normal forecast ensemble members and the 52nd higher resolution forecast
"""
function forecast_ensembles(lat::Real, lon::Real; return_format::Symbol = :csv)
parameters = Dict(:lat => lat, :lon => lon, :return_format => return_format)
forecast_ensembles(parameters)
end
"""
forecast_ensembles(lat::Real, lon::Real, date::AbstractString; return_format::Symbol = :csv)
returns a timeseries for each of the 51 normal forecast ensemble members and the 52nd higher resolution forecast
"""
function forecast_ensembles(
lat::Real,
lon::Real,
date::AbstractString;
return_format::Symbol = :csv,
)
if occursin("-", date)
date = replace(date, "-" => "")
end
parameters = Dict(
:lat => lat,
:lon => lon,
:date => date,
:return_format => return_format,
)
forecast_ensembles(parameters)
end
"""
forecast_ensembles(lat::Real, lon::Real, date::TimeType; return_format::Symbol = :csv)
returns a timeseries for each of the 51 normal forecast ensemble members and the 52nd higher resolution forecast
"""
function forecast_ensembles(
lat::Real,
lon::Real,
date::TimeType;
return_format::Symbol = :csv,
)
datestr = Dates.format(date, "yyyymmdd")
parameters = Dict(
:lat => lat,
:lon => lon,
:date => datestr,
:return_format => return_format,
)
forecast_ensembles(parameters)
end
"""
forecast_records(parameters::AbstractDict{Symbol,<:Any})
retrieves the rolling record of the mean of the forecasted streamflow during the
first 24 hours of each day's forecast. That is, each day day after the streamflow
forecasts are computed, the average of first 8 of the 3-hour timesteps are recorded to a csv.
"""
function forecast_records(parameters::AbstractDict{Symbol,<:Any})
endpoint = "ForecastRecords"
r = _make_request(baseurl, endpoint, parameters)
_read_response(r, parameters[:return_format], with_dates = true)
end
"""
forecast_records(reach_id::Int; return_format::Symbol = :csv)
retrieves the rolling record of the mean of the forecasted streamflow during the
first 24 hours of each day's forecast. That is, each day day after the streamflow
forecasts are computed, the average of first 8 of the 3-hour timesteps are recorded to a csv.
"""
function forecast_records(reach_id::Int; return_format::Symbol = :csv)
parameters = Dict(:reach_id => reach_id, :return_format => return_format)
forecast_records(parameters)
end
"""
forecast_records(lat::Real, lon::Real; return_format::Symbol = :csv)
retrieves the rolling record of the mean of the forecasted streamflow during the
first 24 hours of each day's forecast. That is, each day day after the streamflow
forecasts are computed, the average of first 8 of the 3-hour timesteps are recorded to a csv.
"""
function forecast_records(lat::Real, lon::Real; return_format::Symbol = :csv)
parameters = Dict(:lat => lat, :lon => lon, :return_format => return_format)
forecast_records(parameters)
end
"""
forecast_records(reach_id::Int, start_date::TimeType, end_date::TimeType; return_format::Symbol = :csv,)
retrieves the rolling record of the mean of the forecasted streamflow during the
first 24 hours of each day's forecast. That is, each day day after the streamflow
forecasts are computed, the average of first 8 of the 3-hour timesteps are recorded to a csv.
"""
function forecast_records(
reach_id::Int,
start_date::TimeType,
end_date::TimeType;
return_format::Symbol = :csv,
)
start_datestr = Dates.format(start_date, "yyyymmdd")
end_datestr = Dates.format(end_date, "yyyymmdd")
parameters = Dict(
:reach_id => reach_id,
:start_date => start_datestr,
:end_date => end_datestr,
:return_format => return_format,
)
forecast_records(parameters)
end
"""
forecast_records(reach_id::Int, start_date::AbstractString, end_date::AbstractString; return_format::Symbol = :csv,)
retrieves the rolling record of the mean of the forecasted streamflow during the
first 24 hours of each day's forecast. That is, each day day after the streamflow
forecasts are computed, the average of first 8 of the 3-hour timesteps are recorded to a csv.
"""
function forecast_records(
reach_id::Int,
start_date::AbstractString,
end_date::AbstractString;
return_format::Symbol = :csv,
)
if occursin("-", start_date)
start_date = replace(start_date, "-" => "")
end
if occursin("-", end_date)
end_date = replace(end_date, "-" => "")
end
parameters = Dict(
:reach_id => reach_id,
:start_date => start_date,
:end_date => end_date,
:return_format => return_format,
)
forecast_records(parameters)
end
"""
forecast_records(lat::Real, lon::Real, start_date::TimeType, end_date::TimeType; return_format::Symbol = :csv,)
retrieves the rolling record of the mean of the forecasted streamflow during the
first 24 hours of each day's forecast. That is, each day day after the streamflow
forecasts are computed, the average of first 8 of the 3-hour timesteps are recorded to a csv.
"""
function forecast_records(
lat::Real,
lon::Real,
start_date::TimeType,
end_date::TimeType;
return_format::Symbol = :csv,
)
start_datestr = Dates.format(start_date, "yyyymmdd")
end_datestr = Dates.format(end_date, "yyyymmdd")
parameters = Dict(
:lat => lat,
:lon => lon,
:start_date => start_datestr,
:end_date => end_datestr,
:return_format => return_format,
)
forecast_records(parameters)
end
"""
forecast_records(lat::Real, lon::Real, start_date::AbstractString, end_date::AbstractString; return_format::Symbol = :csv,)
retrieves the rolling record of the mean of the forecasted streamflow during the
first 24 hours of each day's forecast. That is, each day day after the streamflow
forecasts are computed, the average of first 8 of the 3-hour timesteps are recorded to a csv.
"""
function forecast_records(
lat::Real,
lon::Real,
start_date::AbstractString,
end_date::AbstractString;
return_format::Symbol = :csv,
)
if occursin("-", start_date)
start_date = replace(start_date, "-" => "")
end
if occursin("-", end_date)
end_date = replace(end_date, "-" => "")
end
parameters = Dict(
:lat => lat,
:lon => lon,
:start_date => start_date,
:end_date => end_date,
:return_format => return_format,
)
forecast_records(parameters)
end
"""
forecast_warnings(parameters::AbstractDict{Symbol,<:Any})
returns a csv created to summarize the potential return period level flow events
coming to the reaches in a specified region. The CSV contains a column for the
reach_id, lat of the reach, lon of the reach, maximum forecasted flow in the next
15 day forecast, and a column for each of the return periods (2, 10, 20, 25, 50, 100)
which will contain the date when the forecast is first expected to pass that threshold
"""
function forecast_warnings(parameters::AbstractDict{Symbol,<:Any})
endpoint = "ForecastWarnings"
r = _make_request(baseurl, endpoint, parameters)
_read_response(r, parameters[:return_format])
end
"""
forecast_warnings(; return_format::Symbol = :csv)
returns a csv created to summarize the potential return period level flow events
coming to the reaches in a specified region. The CSV contains a column for the
reach_id, lat of the reach, lon of the reach, maximum forecasted flow in the next
15 day forecast, and a column for each of the return periods (2, 10, 20, 25, 50, 100)
which will contain the date when the forecast is first expected to pass that threshold
"""
function forecast_warnings(; return_format::Symbol = :csv)
parameters = Dict(:return_format => return_format)
forecast_warnings(parameters)
end
"""
forecast_warnings(date::TimeType; return_format::Symbol = :csv)
returns a csv created to summarize the potential return period level flow events
coming to the reaches in a specified region. The CSV contains a column for the
reach_id, lat of the reach, lon of the reach, maximum forecasted flow in the next
15 day forecast, and a column for each of the return periods (2, 10, 20, 25, 50, 100)
which will contain the date when the forecast is first expected to pass that threshold
"""
function forecast_warnings(date::TimeType; return_format::Symbol = :csv)
datestr = Dates.format(date, "yyyymmdd")
parameters = Dict(:date => datestr, :return_format => return_format)
forecast_warnings(parameters)
end
"""
forecast_warnings(region::AbstractString; return_format::Symbol = :csv)
returns a csv created to summarize the potential return period level flow events
coming to the reaches in a specified region. The CSV contains a column for the
reach_id, lat of the reach, lon of the reach, maximum forecasted flow in the next
15 day forecast, and a column for each of the return periods (2, 10, 20, 25, 50, 100)
which will contain the date when the forecast is first expected to pass that threshold
"""
function forecast_warnings(region::AbstractString; return_format::Symbol = :csv)
parameters = Dict(:region => region, :return_format => return_format)
forecast_warnings(parameters)
end
"""
forecast_warnings(region::AbstractString, date::TimeType; return_format::Symbol = :csv)
returns a csv created to summarize the potential return period level flow events
coming to the reaches in a specified region. The CSV contains a column for the
reach_id, lat of the reach, lon of the reach, maximum forecasted flow in the next
15 day forecast, and a column for each of the return periods (2, 10, 20, 25, 50, 100)
which will contain the date when the forecast is first expected to pass that threshold
"""
function forecast_warnings(
region::AbstractString,
date::TimeType;
return_format::Symbol = :csv,
)
datestr = Dates.format(date, "yyyymmdd")
parameters = Dict(
:region => region,
:date => datestr,
:return_format => return_format,
)
forecast_warnings(parameters)
end
"""
forecast_warnings(region::AbstractString, date::AbstractString; return_format::Symbol = :csv)
returns a csv created to summarize the potential return period level flow events
coming to the reaches in a specified region. The CSV contains a column for the
reach_id, lat of the reach, lon of the reach, maximum forecasted flow in the next
15 day forecast, and a column for each of the return periods (2, 10, 20, 25, 50, 100)
which will contain the date when the forecast is first expected to pass that threshold
"""
function forecast_warnings(
region::AbstractString,
date::AbstractString;
return_format::Symbol = :csv,
)
if occursin("-", date)
date = replace(date, "-" => "")
end
parameters = Dict(
region::AbstractString,
:date => date,
:return_format => return_format,
)
forecast_warnings(parameters)
end
| [
2,
2393,
7268,
5050,
329,
20623,
11092,
27785,
422,
402,
4720,
38,
5439,
19416,
198,
37811,
198,
220,
220,
220,
11092,
62,
34242,
7,
17143,
7307,
3712,
23839,
35,
713,
90,
13940,
23650,
11,
27,
25,
7149,
30072,
198,
198,
7783,
82,
7869,
10488,
422,
6885,
11092,
34549,
1866,
13,
198,
37811,
198,
8818,
11092,
62,
34242,
7,
17143,
7307,
3712,
23839,
35,
713,
90,
13940,
23650,
11,
27,
25,
7149,
30072,
198,
220,
220,
220,
36123,
796,
366,
16351,
2701,
29668,
1,
628,
220,
220,
220,
374,
796,
4808,
15883,
62,
25927,
7,
8692,
6371,
11,
36123,
11,
10007,
8,
628,
220,
220,
220,
4808,
961,
62,
26209,
7,
81,
11,
10007,
58,
25,
7783,
62,
18982,
4357,
351,
62,
19581,
796,
2081,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
34242,
7,
16250,
62,
312,
3712,
5317,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
7869,
10488,
422,
6885,
11092,
34549,
1866,
13,
198,
37811,
198,
8818,
11092,
62,
34242,
7,
16250,
62,
312,
3712,
5317,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
220,
220,
220,
10007,
796,
360,
713,
7,
25,
16250,
62,
312,
5218,
3151,
62,
312,
11,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
8,
628,
220,
220,
220,
11092,
62,
34242,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
34242,
7,
16250,
62,
312,
3712,
5317,
11,
4475,
3712,
23839,
10100,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
7869,
10488,
422,
6885,
11092,
34549,
1866,
13,
198,
37811,
198,
8818,
11092,
62,
34242,
7,
198,
220,
220,
220,
3151,
62,
312,
3712,
5317,
11,
198,
220,
220,
220,
3128,
3712,
23839,
10100,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
16250,
62,
312,
5218,
3151,
62,
312,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
4475,
5218,
3128,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
34242,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
34242,
7,
16250,
62,
312,
3712,
5317,
11,
4475,
3712,
7575,
6030,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
7869,
10488,
422,
6885,
11092,
34549,
1866,
13,
198,
37811,
198,
8818,
11092,
62,
34242,
7,
198,
220,
220,
220,
3151,
62,
312,
3712,
5317,
11,
198,
220,
220,
220,
3128,
3712,
7575,
6030,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
4818,
395,
81,
796,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
44712,
13,
18982,
7,
4475,
11,
366,
26314,
26314,
12,
76,
12,
67,
4943,
198,
220,
220,
220,
4929,
198,
220,
220,
220,
220,
220,
220,
220,
44712,
13,
18982,
7,
4475,
11,
366,
22556,
22556,
3020,
1860,
4943,
198,
220,
220,
220,
886,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
16250,
62,
312,
5218,
3151,
62,
312,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
4475,
5218,
4818,
395,
81,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
34242,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
34242,
7,
15460,
3712,
15633,
11,
300,
261,
3712,
15633,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
7869,
10488,
422,
6885,
11092,
34549,
1866,
13,
198,
37811,
198,
8818,
11092,
62,
34242,
7,
15460,
3712,
15633,
11,
300,
261,
3712,
15633,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
220,
220,
220,
10007,
796,
360,
713,
7,
25,
15460,
5218,
3042,
11,
1058,
14995,
5218,
300,
261,
11,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
8,
628,
220,
220,
220,
11092,
62,
34242,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
34242,
7,
15460,
3712,
15633,
11,
300,
261,
3712,
15633,
11,
3128,
3712,
23839,
10100,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
7869,
10488,
422,
6885,
11092,
34549,
1866,
13,
198,
37811,
198,
8818,
11092,
62,
34242,
7,
198,
220,
220,
220,
3042,
3712,
15633,
11,
198,
220,
220,
220,
300,
261,
3712,
15633,
11,
198,
220,
220,
220,
3128,
3712,
23839,
10100,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
611,
8833,
259,
7203,
12,
1600,
3128,
8,
198,
220,
220,
220,
220,
220,
220,
220,
3128,
796,
6330,
7,
4475,
11,
366,
21215,
5218,
366,
4943,
198,
220,
220,
220,
886,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
15460,
5218,
3042,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
14995,
5218,
300,
261,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
4475,
5218,
3128,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
34242,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
34242,
7,
15460,
3712,
15633,
11,
300,
261,
3712,
15633,
11,
3128,
3712,
7575,
6030,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
7869,
10488,
422,
6885,
11092,
34549,
1866,
13,
198,
37811,
198,
8818,
11092,
62,
34242,
7,
198,
220,
220,
220,
3042,
3712,
15633,
11,
198,
220,
220,
220,
300,
261,
3712,
15633,
11,
198,
220,
220,
220,
3128,
3712,
7575,
6030,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
4818,
395,
81,
796,
44712,
13,
18982,
7,
4475,
11,
366,
22556,
22556,
3020,
1860,
4943,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
15460,
5218,
3042,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
14995,
5218,
300,
261,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
4475,
5218,
4818,
395,
81,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
34242,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
17143,
7307,
3712,
23839,
35,
713,
90,
13940,
23650,
11,
27,
25,
7149,
30072,
198,
198,
7783,
82,
257,
1661,
10640,
329,
1123,
286,
262,
6885,
3487,
11092,
34549,
1866,
290,
262,
6740,
358,
2440,
6323,
11092,
198,
37811,
198,
8818,
11092,
62,
1072,
2022,
829,
7,
17143,
7307,
3712,
23839,
35,
713,
90,
13940,
23650,
11,
27,
25,
7149,
30072,
198,
220,
220,
220,
36123,
796,
366,
16351,
2701,
4834,
4428,
829,
1,
628,
220,
220,
220,
374,
796,
4808,
15883,
62,
25927,
7,
8692,
6371,
11,
36123,
11,
10007,
8,
628,
220,
220,
220,
4808,
961,
62,
26209,
7,
81,
11,
10007,
58,
25,
7783,
62,
18982,
4357,
351,
62,
19581,
796,
2081,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
16250,
62,
312,
3712,
5317,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
257,
1661,
10640,
329,
1123,
286,
262,
6885,
3487,
11092,
34549,
1866,
290,
262,
6740,
358,
2440,
6323,
11092,
198,
37811,
198,
8818,
11092,
62,
1072,
2022,
829,
7,
16250,
62,
312,
3712,
5317,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
220,
220,
220,
10007,
796,
360,
713,
7,
25,
16250,
62,
312,
5218,
3151,
62,
312,
11,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
8,
628,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
16250,
62,
312,
3712,
5317,
11,
3128,
3712,
23839,
10100,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
257,
1661,
10640,
329,
1123,
286,
262,
6885,
3487,
11092,
34549,
1866,
290,
262,
6740,
358,
2440,
6323,
11092,
198,
37811,
198,
8818,
11092,
62,
1072,
2022,
829,
7,
198,
220,
220,
220,
3151,
62,
312,
3712,
5317,
11,
198,
220,
220,
220,
3128,
3712,
23839,
10100,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
611,
8833,
259,
7203,
12,
1600,
3128,
8,
198,
220,
220,
220,
220,
220,
220,
220,
3128,
796,
6330,
7,
4475,
11,
366,
21215,
5218,
366,
4943,
198,
220,
220,
220,
886,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
16250,
62,
312,
5218,
3151,
62,
312,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
4475,
5218,
3128,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
16250,
62,
312,
3712,
5317,
11,
3128,
3712,
7575,
6030,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
257,
1661,
10640,
329,
1123,
286,
262,
6885,
3487,
11092,
34549,
1866,
290,
262,
6740,
358,
2440,
6323,
11092,
198,
37811,
198,
8818,
11092,
62,
1072,
2022,
829,
7,
198,
220,
220,
220,
3151,
62,
312,
3712,
5317,
11,
198,
220,
220,
220,
3128,
3712,
7575,
6030,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
4818,
395,
81,
796,
44712,
13,
18982,
7,
4475,
11,
366,
22556,
22556,
3020,
1860,
4943,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
16250,
62,
312,
5218,
3151,
62,
312,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
4475,
5218,
4818,
395,
81,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
15460,
3712,
15633,
11,
300,
261,
3712,
15633,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
257,
1661,
10640,
329,
1123,
286,
262,
6885,
3487,
11092,
34549,
1866,
290,
262,
6740,
358,
2440,
6323,
11092,
198,
37811,
198,
8818,
11092,
62,
1072,
2022,
829,
7,
15460,
3712,
15633,
11,
300,
261,
3712,
15633,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
220,
220,
220,
10007,
796,
360,
713,
7,
25,
15460,
5218,
3042,
11,
1058,
14995,
5218,
300,
261,
11,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
8,
628,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
15460,
3712,
15633,
11,
300,
261,
3712,
15633,
11,
3128,
3712,
23839,
10100,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
257,
1661,
10640,
329,
1123,
286,
262,
6885,
3487,
11092,
34549,
1866,
290,
262,
6740,
358,
2440,
6323,
11092,
198,
37811,
198,
8818,
11092,
62,
1072,
2022,
829,
7,
198,
220,
220,
220,
3042,
3712,
15633,
11,
198,
220,
220,
220,
300,
261,
3712,
15633,
11,
198,
220,
220,
220,
3128,
3712,
23839,
10100,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
611,
8833,
259,
7203,
12,
1600,
3128,
8,
198,
220,
220,
220,
220,
220,
220,
220,
3128,
796,
6330,
7,
4475,
11,
366,
21215,
5218,
366,
4943,
198,
220,
220,
220,
886,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
15460,
5218,
3042,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
14995,
5218,
300,
261,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
4475,
5218,
3128,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
15460,
3712,
15633,
11,
300,
261,
3712,
15633,
11,
3128,
3712,
7575,
6030,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
257,
1661,
10640,
329,
1123,
286,
262,
6885,
3487,
11092,
34549,
1866,
290,
262,
6740,
358,
2440,
6323,
11092,
198,
37811,
198,
8818,
11092,
62,
1072,
2022,
829,
7,
198,
220,
220,
220,
3042,
3712,
15633,
11,
198,
220,
220,
220,
300,
261,
3712,
15633,
11,
198,
220,
220,
220,
3128,
3712,
7575,
6030,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
4818,
395,
81,
796,
44712,
13,
18982,
7,
4475,
11,
366,
22556,
22556,
3020,
1860,
4943,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
15460,
5218,
3042,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
14995,
5218,
300,
261,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
4475,
5218,
4818,
395,
81,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
1072,
2022,
829,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
8344,
3669,
7,
17143,
7307,
3712,
23839,
35,
713,
90,
13940,
23650,
11,
27,
25,
7149,
30072,
198,
198,
1186,
5034,
1158,
262,
10708,
1700,
286,
262,
1612,
286,
262,
11092,
276,
4269,
11125,
1141,
262,
198,
11085,
1987,
2250,
286,
1123,
1110,
338,
11092,
13,
1320,
318,
11,
1123,
1110,
1110,
706,
262,
4269,
11125,
198,
754,
40924,
389,
29231,
11,
262,
2811,
286,
717,
807,
286,
262,
513,
12,
9769,
4628,
395,
25386,
389,
6264,
284,
257,
269,
21370,
13,
198,
37811,
198,
8818,
11092,
62,
8344,
3669,
7,
17143,
7307,
3712,
23839,
35,
713,
90,
13940,
23650,
11,
27,
25,
7149,
30072,
198,
220,
220,
220,
36123,
796,
366,
16351,
2701,
6690,
3669,
1,
628,
220,
220,
220,
374,
796,
4808,
15883,
62,
25927,
7,
8692,
6371,
11,
36123,
11,
10007,
8,
628,
220,
220,
220,
4808,
961,
62,
26209,
7,
81,
11,
10007,
58,
25,
7783,
62,
18982,
4357,
351,
62,
19581,
796,
2081,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
8344,
3669,
7,
16250,
62,
312,
3712,
5317,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
1186,
5034,
1158,
262,
10708,
1700,
286,
262,
1612,
286,
262,
11092,
276,
4269,
11125,
1141,
262,
198,
11085,
1987,
2250,
286,
1123,
1110,
338,
11092,
13,
1320,
318,
11,
1123,
1110,
1110,
706,
262,
4269,
11125,
198,
754,
40924,
389,
29231,
11,
262,
2811,
286,
717,
807,
286,
262,
513,
12,
9769,
4628,
395,
25386,
389,
6264,
284,
257,
269,
21370,
13,
198,
37811,
198,
8818,
11092,
62,
8344,
3669,
7,
16250,
62,
312,
3712,
5317,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
220,
220,
220,
10007,
796,
360,
713,
7,
25,
16250,
62,
312,
5218,
3151,
62,
312,
11,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
8,
628,
220,
220,
220,
11092,
62,
8344,
3669,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
8344,
3669,
7,
15460,
3712,
15633,
11,
300,
261,
3712,
15633,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
1186,
5034,
1158,
262,
10708,
1700,
286,
262,
1612,
286,
262,
11092,
276,
4269,
11125,
1141,
262,
198,
11085,
1987,
2250,
286,
1123,
1110,
338,
11092,
13,
1320,
318,
11,
1123,
1110,
1110,
706,
262,
4269,
11125,
198,
754,
40924,
389,
29231,
11,
262,
2811,
286,
717,
807,
286,
262,
513,
12,
9769,
4628,
395,
25386,
389,
6264,
284,
257,
269,
21370,
13,
198,
37811,
198,
8818,
11092,
62,
8344,
3669,
7,
15460,
3712,
15633,
11,
300,
261,
3712,
15633,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
220,
220,
220,
10007,
796,
360,
713,
7,
25,
15460,
5218,
3042,
11,
1058,
14995,
5218,
300,
261,
11,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
8,
628,
220,
220,
220,
11092,
62,
8344,
3669,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
8344,
3669,
7,
16250,
62,
312,
3712,
5317,
11,
923,
62,
4475,
3712,
7575,
6030,
11,
886,
62,
4475,
3712,
7575,
6030,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
35751,
198,
198,
1186,
5034,
1158,
262,
10708,
1700,
286,
262,
1612,
286,
262,
11092,
276,
4269,
11125,
1141,
262,
198,
11085,
1987,
2250,
286,
1123,
1110,
338,
11092,
13,
1320,
318,
11,
1123,
1110,
1110,
706,
262,
4269,
11125,
198,
754,
40924,
389,
29231,
11,
262,
2811,
286,
717,
807,
286,
262,
513,
12,
9769,
4628,
395,
25386,
389,
6264,
284,
257,
269,
21370,
13,
198,
37811,
198,
8818,
11092,
62,
8344,
3669,
7,
198,
220,
220,
220,
3151,
62,
312,
3712,
5317,
11,
198,
220,
220,
220,
923,
62,
4475,
3712,
7575,
6030,
11,
198,
220,
220,
220,
886,
62,
4475,
3712,
7575,
6030,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
923,
62,
19608,
395,
81,
796,
44712,
13,
18982,
7,
9688,
62,
4475,
11,
366,
22556,
22556,
3020,
1860,
4943,
198,
220,
220,
220,
886,
62,
19608,
395,
81,
796,
44712,
13,
18982,
7,
437,
62,
4475,
11,
366,
22556,
22556,
3020,
1860,
4943,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
16250,
62,
312,
5218,
3151,
62,
312,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
9688,
62,
4475,
5218,
923,
62,
19608,
395,
81,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
437,
62,
4475,
5218,
886,
62,
19608,
395,
81,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
8344,
3669,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
8344,
3669,
7,
16250,
62,
312,
3712,
5317,
11,
923,
62,
4475,
3712,
23839,
10100,
11,
886,
62,
4475,
3712,
23839,
10100,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
35751,
198,
198,
1186,
5034,
1158,
262,
10708,
1700,
286,
262,
1612,
286,
262,
11092,
276,
4269,
11125,
1141,
262,
198,
11085,
1987,
2250,
286,
1123,
1110,
338,
11092,
13,
1320,
318,
11,
1123,
1110,
1110,
706,
262,
4269,
11125,
198,
754,
40924,
389,
29231,
11,
262,
2811,
286,
717,
807,
286,
262,
513,
12,
9769,
4628,
395,
25386,
389,
6264,
284,
257,
269,
21370,
13,
198,
37811,
198,
8818,
11092,
62,
8344,
3669,
7,
198,
220,
220,
220,
3151,
62,
312,
3712,
5317,
11,
198,
220,
220,
220,
923,
62,
4475,
3712,
23839,
10100,
11,
198,
220,
220,
220,
886,
62,
4475,
3712,
23839,
10100,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
611,
8833,
259,
7203,
12,
1600,
923,
62,
4475,
8,
198,
220,
220,
220,
220,
220,
220,
220,
923,
62,
4475,
796,
6330,
7,
9688,
62,
4475,
11,
366,
21215,
5218,
366,
4943,
198,
220,
220,
220,
886,
198,
220,
220,
220,
611,
8833,
259,
7203,
12,
1600,
886,
62,
4475,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
62,
4475,
796,
6330,
7,
437,
62,
4475,
11,
366,
21215,
5218,
366,
4943,
198,
220,
220,
220,
886,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
16250,
62,
312,
5218,
3151,
62,
312,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
9688,
62,
4475,
5218,
923,
62,
4475,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
437,
62,
4475,
5218,
886,
62,
4475,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
8344,
3669,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
8344,
3669,
7,
15460,
3712,
15633,
11,
300,
261,
3712,
15633,
11,
923,
62,
4475,
3712,
7575,
6030,
11,
886,
62,
4475,
3712,
7575,
6030,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
35751,
198,
198,
1186,
5034,
1158,
262,
10708,
1700,
286,
262,
1612,
286,
262,
11092,
276,
4269,
11125,
1141,
262,
198,
11085,
1987,
2250,
286,
1123,
1110,
338,
11092,
13,
1320,
318,
11,
1123,
1110,
1110,
706,
262,
4269,
11125,
198,
754,
40924,
389,
29231,
11,
262,
2811,
286,
717,
807,
286,
262,
513,
12,
9769,
4628,
395,
25386,
389,
6264,
284,
257,
269,
21370,
13,
198,
37811,
198,
8818,
11092,
62,
8344,
3669,
7,
198,
220,
220,
220,
3042,
3712,
15633,
11,
198,
220,
220,
220,
300,
261,
3712,
15633,
11,
198,
220,
220,
220,
923,
62,
4475,
3712,
7575,
6030,
11,
198,
220,
220,
220,
886,
62,
4475,
3712,
7575,
6030,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
923,
62,
19608,
395,
81,
796,
44712,
13,
18982,
7,
9688,
62,
4475,
11,
366,
22556,
22556,
3020,
1860,
4943,
198,
220,
220,
220,
886,
62,
19608,
395,
81,
796,
44712,
13,
18982,
7,
437,
62,
4475,
11,
366,
22556,
22556,
3020,
1860,
4943,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
15460,
5218,
3042,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
14995,
5218,
300,
261,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
9688,
62,
4475,
5218,
923,
62,
19608,
395,
81,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
437,
62,
4475,
5218,
886,
62,
19608,
395,
81,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
8344,
3669,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
8344,
3669,
7,
15460,
3712,
15633,
11,
300,
261,
3712,
15633,
11,
923,
62,
4475,
3712,
23839,
10100,
11,
886,
62,
4475,
3712,
23839,
10100,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
35751,
198,
198,
1186,
5034,
1158,
262,
10708,
1700,
286,
262,
1612,
286,
262,
11092,
276,
4269,
11125,
1141,
262,
198,
11085,
1987,
2250,
286,
1123,
1110,
338,
11092,
13,
1320,
318,
11,
1123,
1110,
1110,
706,
262,
4269,
11125,
198,
754,
40924,
389,
29231,
11,
262,
2811,
286,
717,
807,
286,
262,
513,
12,
9769,
4628,
395,
25386,
389,
6264,
284,
257,
269,
21370,
13,
198,
37811,
198,
8818,
11092,
62,
8344,
3669,
7,
198,
220,
220,
220,
3042,
3712,
15633,
11,
198,
220,
220,
220,
300,
261,
3712,
15633,
11,
198,
220,
220,
220,
923,
62,
4475,
3712,
23839,
10100,
11,
198,
220,
220,
220,
886,
62,
4475,
3712,
23839,
10100,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
611,
8833,
259,
7203,
12,
1600,
923,
62,
4475,
8,
198,
220,
220,
220,
220,
220,
220,
220,
923,
62,
4475,
796,
6330,
7,
9688,
62,
4475,
11,
366,
21215,
5218,
366,
4943,
198,
220,
220,
220,
886,
198,
220,
220,
220,
611,
8833,
259,
7203,
12,
1600,
886,
62,
4475,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
62,
4475,
796,
6330,
7,
437,
62,
4475,
11,
366,
21215,
5218,
366,
4943,
198,
220,
220,
220,
886,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
15460,
5218,
3042,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
14995,
5218,
300,
261,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
9688,
62,
4475,
5218,
923,
62,
4475,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
437,
62,
4475,
5218,
886,
62,
4475,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
8344,
3669,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
40539,
654,
7,
17143,
7307,
3712,
23839,
35,
713,
90,
13940,
23650,
11,
27,
25,
7149,
30072,
198,
198,
7783,
82,
257,
269,
21370,
2727,
284,
35743,
262,
2785,
1441,
2278,
1241,
5202,
2995,
198,
4976,
284,
262,
12229,
287,
257,
7368,
3814,
13,
383,
44189,
4909,
257,
5721,
329,
262,
198,
16250,
62,
312,
11,
3042,
286,
262,
3151,
11,
300,
261,
286,
262,
3151,
11,
5415,
11092,
276,
5202,
287,
262,
1306,
198,
1314,
1110,
11092,
11,
290,
257,
5721,
329,
1123,
286,
262,
1441,
9574,
357,
17,
11,
838,
11,
1160,
11,
1679,
11,
2026,
11,
1802,
8,
198,
4758,
481,
3994,
262,
3128,
618,
262,
11092,
318,
717,
2938,
284,
1208,
326,
11387,
198,
37811,
198,
8818,
11092,
62,
40539,
654,
7,
17143,
7307,
3712,
23839,
35,
713,
90,
13940,
23650,
11,
27,
25,
7149,
30072,
198,
220,
220,
220,
36123,
796,
366,
16351,
2701,
54,
1501,
654,
1,
628,
220,
220,
220,
374,
796,
4808,
15883,
62,
25927,
7,
8692,
6371,
11,
36123,
11,
10007,
8,
628,
220,
220,
220,
4808,
961,
62,
26209,
7,
81,
11,
10007,
58,
25,
7783,
62,
18982,
12962,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
40539,
654,
7,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
257,
269,
21370,
2727,
284,
35743,
262,
2785,
1441,
2278,
1241,
5202,
2995,
198,
4976,
284,
262,
12229,
287,
257,
7368,
3814,
13,
383,
44189,
4909,
257,
5721,
329,
262,
198,
16250,
62,
312,
11,
3042,
286,
262,
3151,
11,
300,
261,
286,
262,
3151,
11,
5415,
11092,
276,
5202,
287,
262,
1306,
198,
1314,
1110,
11092,
11,
290,
257,
5721,
329,
1123,
286,
262,
1441,
9574,
357,
17,
11,
838,
11,
1160,
11,
1679,
11,
2026,
11,
1802,
8,
198,
4758,
481,
3994,
262,
3128,
618,
262,
11092,
318,
717,
2938,
284,
1208,
326,
11387,
198,
37811,
198,
8818,
11092,
62,
40539,
654,
7,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
220,
220,
220,
10007,
796,
360,
713,
7,
25,
7783,
62,
18982,
5218,
1441,
62,
18982,
8,
628,
220,
220,
220,
11092,
62,
40539,
654,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
40539,
654,
7,
4475,
3712,
7575,
6030,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
257,
269,
21370,
2727,
284,
35743,
262,
2785,
1441,
2278,
1241,
5202,
2995,
198,
4976,
284,
262,
12229,
287,
257,
7368,
3814,
13,
383,
44189,
4909,
257,
5721,
329,
262,
198,
16250,
62,
312,
11,
3042,
286,
262,
3151,
11,
300,
261,
286,
262,
3151,
11,
5415,
11092,
276,
5202,
287,
262,
1306,
198,
1314,
1110,
11092,
11,
290,
257,
5721,
329,
1123,
286,
262,
1441,
9574,
357,
17,
11,
838,
11,
1160,
11,
1679,
11,
2026,
11,
1802,
8,
198,
4758,
481,
3994,
262,
3128,
618,
262,
11092,
318,
717,
2938,
284,
1208,
326,
11387,
198,
37811,
198,
8818,
11092,
62,
40539,
654,
7,
4475,
3712,
7575,
6030,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
220,
220,
220,
4818,
395,
81,
796,
44712,
13,
18982,
7,
4475,
11,
366,
22556,
22556,
3020,
1860,
4943,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
25,
4475,
5218,
4818,
395,
81,
11,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
8,
628,
220,
220,
220,
11092,
62,
40539,
654,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
40539,
654,
7,
36996,
3712,
23839,
10100,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
257,
269,
21370,
2727,
284,
35743,
262,
2785,
1441,
2278,
1241,
5202,
2995,
198,
4976,
284,
262,
12229,
287,
257,
7368,
3814,
13,
383,
44189,
4909,
257,
5721,
329,
262,
198,
16250,
62,
312,
11,
3042,
286,
262,
3151,
11,
300,
261,
286,
262,
3151,
11,
5415,
11092,
276,
5202,
287,
262,
1306,
198,
1314,
1110,
11092,
11,
290,
257,
5721,
329,
1123,
286,
262,
1441,
9574,
357,
17,
11,
838,
11,
1160,
11,
1679,
11,
2026,
11,
1802,
8,
198,
4758,
481,
3994,
262,
3128,
618,
262,
11092,
318,
717,
2938,
284,
1208,
326,
11387,
198,
37811,
198,
8818,
11092,
62,
40539,
654,
7,
36996,
3712,
23839,
10100,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
220,
220,
220,
10007,
796,
360,
713,
7,
25,
36996,
5218,
3814,
11,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
8,
628,
220,
220,
220,
11092,
62,
40539,
654,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
40539,
654,
7,
36996,
3712,
23839,
10100,
11,
3128,
3712,
7575,
6030,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
257,
269,
21370,
2727,
284,
35743,
262,
2785,
1441,
2278,
1241,
5202,
2995,
198,
4976,
284,
262,
12229,
287,
257,
7368,
3814,
13,
383,
44189,
4909,
257,
5721,
329,
262,
198,
16250,
62,
312,
11,
3042,
286,
262,
3151,
11,
300,
261,
286,
262,
3151,
11,
5415,
11092,
276,
5202,
287,
262,
1306,
198,
1314,
1110,
11092,
11,
290,
257,
5721,
329,
1123,
286,
262,
1441,
9574,
357,
17,
11,
838,
11,
1160,
11,
1679,
11,
2026,
11,
1802,
8,
198,
4758,
481,
3994,
262,
3128,
618,
262,
11092,
318,
717,
2938,
284,
1208,
326,
11387,
198,
37811,
198,
8818,
11092,
62,
40539,
654,
7,
198,
220,
220,
220,
3814,
3712,
23839,
10100,
11,
198,
220,
220,
220,
3128,
3712,
7575,
6030,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
4818,
395,
81,
796,
44712,
13,
18982,
7,
4475,
11,
366,
22556,
22556,
3020,
1860,
4943,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
36996,
5218,
3814,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
4475,
5218,
4818,
395,
81,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
40539,
654,
7,
17143,
7307,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
11092,
62,
40539,
654,
7,
36996,
3712,
23839,
10100,
11,
3128,
3712,
23839,
10100,
26,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
8,
198,
198,
7783,
82,
257,
269,
21370,
2727,
284,
35743,
262,
2785,
1441,
2278,
1241,
5202,
2995,
198,
4976,
284,
262,
12229,
287,
257,
7368,
3814,
13,
383,
44189,
4909,
257,
5721,
329,
262,
198,
16250,
62,
312,
11,
3042,
286,
262,
3151,
11,
300,
261,
286,
262,
3151,
11,
5415,
11092,
276,
5202,
287,
262,
1306,
198,
1314,
1110,
11092,
11,
290,
257,
5721,
329,
1123,
286,
262,
1441,
9574,
357,
17,
11,
838,
11,
1160,
11,
1679,
11,
2026,
11,
1802,
8,
198,
4758,
481,
3994,
262,
3128,
618,
262,
11092,
318,
717,
2938,
284,
1208,
326,
11387,
198,
37811,
198,
8818,
11092,
62,
40539,
654,
7,
198,
220,
220,
220,
3814,
3712,
23839,
10100,
11,
198,
220,
220,
220,
3128,
3712,
23839,
10100,
26,
198,
220,
220,
220,
1441,
62,
18982,
3712,
13940,
23650,
796,
1058,
40664,
11,
198,
8,
198,
220,
220,
220,
611,
8833,
259,
7203,
12,
1600,
3128,
8,
198,
220,
220,
220,
220,
220,
220,
220,
3128,
796,
6330,
7,
4475,
11,
366,
21215,
5218,
366,
4943,
198,
220,
220,
220,
886,
628,
220,
220,
220,
10007,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
3814,
3712,
23839,
10100,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
4475,
5218,
3128,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
7783,
62,
18982,
5218,
1441,
62,
18982,
11,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
11092,
62,
40539,
654,
7,
17143,
7307,
8,
198,
437,
198
] | 2.89991 | 5,565 |
import Base.LinAlg:
A_mul_B!, At_mul_B, A_ldiv_B!
import Tulip:
Model, PrimalDualPoint
"""
solve(model, tol, verbose)
Solve model m using an infeasible predictor-corrector Interior-Point algorithm.
# Arguments
- `model::Model`: the optimization model
- `tol::Float64`: numerical tolerance
- `verbose::Int`: 0 means no output, 1 displays log at each iteration
"""
function solve!(
model::Model;
tol::Float64 = 10.0^-8,
verbose::Int = 0
)
model.status = :Built
N_ITER_MAX = 100 # maximum number of IP iterations
niter = 0 # number of IP iterations
# TODO: pre-optimization stuff
F = symbolic_cholesky(model.A) # Symbolic factorization
θ = zeros(model.sol.x)
# X = Array{PrimalDualPoint, 1}()
# TODO: check stopping criterion for possible early termination
# compute starting point
# TODO: decide which starting point
compute_starting_point!(
model.A,
F,
model.sol,
model.b, model.c, model.uind, model.uval
)
# IPM log
if verbose == 1
println(" Itn Primal Obj Dual Obj Prim Inf Dual Inf UBnd Inf\n")
end
# main loop
# push!(X, copy(model.sol))
while niter < N_ITER_MAX
# I. Form and factor Newton System
compute_newton!(
model.A,
model.sol.x,
model.sol.s,
model.sol.w,
model.sol.z,
model.uind,
θ,
F
)
# II. Compute and take step
compute_next_iterate!(model, F)
# III. Book-keeping + display log
# compute residuals
rb = model.A * model.sol.x - model.b
rc = At_mul_B(model.A, model.sol.y) + model.sol.s - model.c
spxpay!(-1.0, rc, model.uind, model.sol.z)
ru = model.sol.x[model.uind] + model.sol.w - model.uval
obj_primal = dot(model.sol.x, model.c)
obj_dual = dot(model.b, model.sol.y) - dot(model.uval, model.sol.z)
niter += 1
# check stopping criterion
eps_p = (norm(rb)) / (1.0 + norm(model.b))
eps_d = (norm(rc)) / (1.0 + norm(model.c))
eps_u = (norm(ru)) / (1.0 + norm(model.uval))
eps_g = abs(obj_primal - obj_dual) / (1.0 + abs(obj_primal))
# if verbose == 1
# print("\teps_p=")
# print(@sprintf("%9.2e", eps_p))
# print("\teps_d=")
# print(@sprintf("%9.2e", eps_d))
# print("\teps_u=")
# print(@sprintf("%9.2e", eps_u))
# print("\teps_g=")
# print(@sprintf("%9.2e", eps_g))
# println("\n")
# end
if verbose == 1
print(@sprintf("%4d", niter)) # iteration count
print(@sprintf("%+18.7e", obj_primal)) # primal objective
print(@sprintf("%+16.7e", obj_dual)) # dual objective
print(@sprintf("%10.2e", norm(rb, Inf))) # primal infeas
print(@sprintf("%9.2e", norm(rc, Inf))) # dual infeas
print(@sprintf("%9.2e", norm(ru, Inf))) # upper bound infeas
print(@sprintf("%9.2e", abs(obj_primal - obj_dual) / (model.nvars + size(model.uind, 1))))
print("\n")
end
if (eps_p < tol) && (eps_u < tol) && (eps_d < tol) && (eps_g < tol)
model.status = :Optimal
end
# push!(X, copy(model.sol))
# check status
if model.status == :Optimal
if verbose == 1
println()
println("Optimal solution found.")
end
return model.status
end
end
#
return model.status
end
"""
compute_starting_point!
Compute a starting point
# Arguments
-`model::Model`
-`F::Factorization`: Cholesky factor of A*A', where A is the constraint matrix
of the optimization problem.
"""
function compute_starting_point!(
A::AbstractMatrix{Tv},
F::Factorization{Tv},
Λ::Tulip.PrimalDualPoint{Tv},
b::StridedVector{Tv},
c::StridedVector{Tv},
uind::StridedVector{Ti},
uval::StridedVector{Tv}
) where{Tv<:Real, Ti<:Integer}
(m, n) = size(A)
p = size(uind, 1)
rhs = - 2 * b
u_ = zeros(n)
spxpay!(1.0, u_, uind, uval)
rhs += A* u_
#==============================#
#
# I. Solve two QPs
#
#==============================#
# Compute x0
v = F \ rhs
copy!(Λ.x, -0.5 * At_mul_B(A, v))
spxpay!(0.5, Λ.x, uind, uval)
# Compute w0
@inbounds for i in 1:p
j = uind[i]
Λ.w[i] = uval[i] - Λ.x[j]
end
# Compute y0
copy!(Λ.y, F \ (A*c))
# Compute s0
copy!(Λ.s, 0.5 * (At_mul_B(A, Λ.y) - c))
# Compute z0
@inbounds for i in 1:p
j = uind[i]
Λ.z[i] = - Λ.s[j]
end
#==============================#
#
# II. Compute correction
#
#==============================#
dp = zero(Tv)
dd = zero(Tv)
@inbounds for i in 1:n
tmp = -1.5 * Λ.x[i]
if tmp > dp
dp = tmp
end
end
@inbounds for i in 1:p
tmp = -1.5 * Λ.w[i]
if tmp > dp
dp = tmp
end
end
@inbounds for i in 1:n
tmp = -1.5 * Λ.s[i]
if tmp > dd
dd = tmp
end
end
@inbounds for i in 1:p
tmp = -1.5 * Λ.z[i]
if tmp > dd
dd = tmp
end
end
tmp = dot(Λ.x + dp, Λ.s + dd) + dot(Λ.w + dp, Λ.z + dd)
dp += 0.5 * tmp / (sum(Λ.s + dd) + sum(Λ.z + dd))
dd += 0.5 * tmp / (sum(Λ.x + dp) + sum(Λ.w + dp))
#==============================#
#
# III. Apply correction
#
#==============================#
@inbounds for i in 1:n
Λ.x[i] += dp
end
@inbounds for i in 1:n
Λ.s[i] += dd
end
@inbounds for i in 1:p
Λ.w[i] += dp
end
@inbounds for i in 1:p
Λ.z[i] += dd
end
# Done
return Λ
end
function compute_next_iterate!(model::Model, F::Factorization)
(x, y, s, w, z) = (model.sol.x, model.sol.y, model.sol.s, model.sol.w, model.sol.z)
(m, n, p) = model.nconstr, model.nvars, size(model.uind, 1)
d_aff = copy(model.sol)
d_cc = copy(model.sol)
# compute residuals
μ = (
(dot(x, s) + dot(w, z))
/ (n + p)
)
rb = (model.A * x) - model.b
rc = At_mul_B(model.A, y) + s - model.c
spxpay!(-1.0, rc, model.uind, model.sol.z)
ru = x[model.uind] + w - model.uval
rxs = x .* s
rwz = w .* z
θ = x ./ s
update_theta!(θ, x, s, z, w, model.uind)
# compute predictor
solve_newton!(
model.A,
θ,
F,
model.sol,
d_aff,
model.uind,
-rb,
-rc,
-ru,
-rxs,
-rwz
)
# compute step length
(α_pa, α_da) = compute_stepsize(model.sol, d_aff)
# update centrality parameter
μ_aff = (
(
dot(x + α_pa * d_aff.x, s + α_da * d_aff.s)
+ dot(w + α_pa * d_aff.w, z + α_da * d_aff.z)
) / (n + p)
)
σ = clamp((μ_aff / μ)^3, 10.0^-12, 1.0 - 10.0^-12) # clamped for numerical stability
# compute corrector
solve_newton!(
model.A,
θ,
F,
model.sol,
d_cc,
model.uind,
zeros(m),
zeros(n),
zeros(p),
σ*μ*ones(n) - d_aff.x .* d_aff.s,
σ*μ*ones(p) - d_aff.w .* d_aff.z
)
# final step size
d = d_aff + d_cc
(α_p, α_d) = compute_stepsize(model.sol, d, damp=0.99995)
# take step
model.sol.x += α_p * d.x
model.sol.y += α_d * d.y
model.sol.s += α_d * d.s
model.sol.w += α_p * d.w
model.sol.z += α_d * d.z
return model.sol
end
"""
symbolic_cholesky
Compute Cholesky factorization of A*A'
"""
function symbolic_cholesky(A::AbstractMatrix{T}) where {T<:Real}
F = Cholesky.cholesky(A, ones(A.n))
return F
end
"""
compute_newton!
Form and factorize the Newton system, using the normal equations.
"""
function compute_newton!(
A::AbstractMatrix{Ta},
x::AbstractVector{Tx},
s::AbstractVector{Ts},
w::AbstractVector{Tw},
z::AbstractVector{Tz},
uind::AbstractVector{Ti},
θ::AbstractVector{T},
F::Factorization{Ta}
) where {Ta<:Real, Tx<:Real, Ts<:Real, Tw<:Real, Tz<:Real, Ti<:Integer, T<:Real}
# Compute Θ = (X^{-1} S + W^{-1} Z)^{-1}
θ = x ./ s
for i in 1:size(uind, 1)
j = uind[i]
θ[j] = 1.0 / (s[j] / x[j] + z[i] / w[i])
end
# Form the normal equations matrix and compute its factorization
Cholesky.cholesky!(A, θ, F)
return θ
end
"""
solve_newton
Solve Newton system with the given right-hand side.
Overwrites the input d
"""
function solve_newton!(
A::AbstractMatrix{Ta},
θ::AbstractVector{T1},
F::Factorization{Ta},
Λ::PrimalDualPoint,
d::PrimalDualPoint,
uind::AbstractVector{Ti},
ξ_b::AbstractVector{T2},
ξ_c::AbstractVector{T3},
ξ_u::AbstractVector{T4},
ξ_xs::AbstractVector{T5},
ξ_wz::AbstractVector{T6},
) where {Ta<:Real, T1<:Real, T2<:Real, T3<:Real, T4<:Real, T5<:Real, T6<:Real, Ti<:Integer}
ξ_tmp = ξ_c - (ξ_xs ./ Λ.x)
ξ_tmp[uind] += (ξ_wz - (Λ.z .* ξ_u)) ./ Λ.w
d.y = F \ (ξ_b + A * (θ .* ξ_tmp))
d.x = θ .* (At_mul_B(A, d.y) - ξ_tmp)
d.z = (Λ.z .* (-ξ_u + d.x[uind]) + ξ_wz) ./ Λ.w
d.s = (ξ_xs - Λ.s .* d.x) ./ Λ.x
d.w = (ξ_wz - Λ.w .* d.z) ./ Λ.z
# # check if system is solved correctly
# rb = ξ_b - A*d.x
# rc = ξ_c - (A'*d.y + d.s)
# rc[uind] += d.z
# ru = ξ_u - (d.x[uind] + d.w)
# rxs = ξ_xs - (Λ.s .* d.x + Λ.x .* d.s)
# rwz = ξ_wz - (Λ.z .* d.w + Λ.w .* d.z)
# println("Residuals\t(normal eqs)")
# println("||rb|| \t", @sprintf("%.6e", maximum(abs.(rb))))
# println("||rc|| \t", @sprintf("%.6e", maximum(abs.(rc))))
# println("||ru|| \t", @sprintf("%.6e", maximum(abs.(ru))))
# println("||rxs|| \t", @sprintf("%.6e", maximum(abs.(rxs))))
# println("||rwz|| \t", @sprintf("%.6e", maximum(abs.(rwz))))
# println()
return d
end
function compute_stepsize(
tx::AbstractVector{T}, tw::AbstractVector{T}, ts::AbstractVector{T}, tz::AbstractVector{T},
dx::AbstractVector{T}, dw::AbstractVector{T}, ds::AbstractVector{T}, dz::AbstractVector{T};
damp=1.0
) where T<:Real
n = size(tx, 1)
p = size(tw, 1)
n == size(ts, 1) || throw(DimensionMismatch("t.s is wrong size"))
p == size(tz, 1) || throw(DimensionMismatch("t.z is wrong size"))
n == size(dx, 1) || throw(DimensionMismatch("d.x is wrong size"))
n == size(ds, 1) || throw(DimensionMismatch("d.s is wrong size"))
p == size(dw, 1) || throw(DimensionMismatch("d.w is wrong size"))
p == size(dz, 1) || throw(DimensionMismatch("d.z is wrong size"))
ap, ad = -1.0, -1.0
@inbounds for i in 1:n
if dx[i] < 0.0
if (tx[i] / dx[i]) > ap
ap = (tx[i] / dx[i])
end
end
end
@inbounds for i in 1:n
if ds[i] < 0.0
if (ts[i] / ds[i]) > ad
ad = (ts[i] / ds[i])
end
end
end
@inbounds for j in 1:p
if dw[j] < 0.0
if (tw[j] / dw[j]) > ap
ap = (tw[j] / dw[j])
end
end
end
@inbounds for j in 1:p
if dz[j] < 0.0
if (tz[j] / dz[j]) > ad
ad = (tz[j] / dz[j])
end
end
end
ap = - damp * ap
ad = - damp * ad
# println("\t(ap, ad) = ", (ap, ad))
return (ap, ad)
end
function compute_stepsize(t::PrimalDualPoint{T}, d::PrimalDualPoint{T}; damp=1.0) where T<:Real
(ap, ad) = compute_stepsize(t.x, t.w, t.s, t.z, d.x, d.w, d.s, d.z, damp=damp)
return (ap, ad)
end
function update_theta!(θ, x, s, z, w, colind)
# only called from within the optimization, so bounds were checked before
for i in 1:size(colind, 1)
j = colind[i]
θ[j] = 1.0 / (s[j] / x[j] + z[i] / w[i])
end
return nothing
end
"""
spxpay!(α, x, y_ind, y_val)
In-place computation of x += α * y, where y = sparse(y_ind, y_val)
# Arguments
"""
function spxpay!(α::Tv, x::AbstractVector{Tv}, y_ind::AbstractVector{Ti}, y_val::AbstractVector{Tv}) where{Ti<:Integer, Tv<:Real}
for i in 1:size(y_ind, 1)
j = y_ind[i]
x[j] = x[j] + α * y_val[i]
end
return nothing
end
| [
11748,
7308,
13,
14993,
2348,
70,
25,
198,
220,
220,
220,
317,
62,
76,
377,
62,
33,
28265,
1629,
62,
76,
377,
62,
33,
11,
317,
62,
335,
452,
62,
33,
0,
198,
198,
11748,
30941,
541,
25,
198,
220,
220,
220,
9104,
11,
37712,
36248,
12727,
628,
198,
37811,
198,
220,
220,
220,
8494,
7,
19849,
11,
284,
75,
11,
15942,
577,
8,
198,
198,
50,
6442,
2746,
285,
1262,
281,
1167,
30412,
856,
41568,
12,
30283,
273,
19614,
12,
12727,
11862,
13,
198,
198,
2,
20559,
2886,
198,
12,
4600,
19849,
3712,
17633,
63,
25,
262,
23989,
2746,
198,
12,
4600,
83,
349,
3712,
43879,
2414,
63,
25,
29052,
15621,
198,
12,
4600,
19011,
577,
3712,
5317,
63,
25,
657,
1724,
645,
5072,
11,
352,
11298,
2604,
379,
1123,
24415,
198,
37811,
198,
8818,
8494,
0,
7,
198,
220,
220,
220,
2746,
3712,
17633,
26,
198,
220,
220,
220,
284,
75,
3712,
43879,
2414,
796,
838,
13,
15,
61,
12,
23,
11,
198,
220,
220,
220,
15942,
577,
3712,
5317,
796,
657,
198,
8,
628,
220,
220,
220,
2746,
13,
13376,
796,
1058,
39582,
628,
220,
220,
220,
399,
62,
2043,
1137,
62,
22921,
796,
1802,
220,
1303,
5415,
1271,
286,
6101,
34820,
198,
220,
220,
220,
299,
2676,
796,
657,
220,
1303,
1271,
286,
6101,
34820,
628,
220,
220,
220,
1303,
16926,
46,
25,
662,
12,
40085,
1634,
3404,
198,
220,
220,
220,
376,
796,
18975,
62,
354,
4316,
2584,
7,
19849,
13,
32,
8,
220,
1303,
41327,
4160,
5766,
1634,
198,
220,
220,
220,
7377,
116,
796,
1976,
27498,
7,
19849,
13,
34453,
13,
87,
8,
628,
220,
220,
220,
1303,
1395,
796,
15690,
90,
23828,
282,
36248,
12727,
11,
352,
92,
3419,
198,
220,
220,
220,
1303,
16926,
46,
25,
2198,
12225,
34054,
329,
1744,
1903,
19883,
628,
220,
220,
220,
1303,
24061,
3599,
966,
198,
220,
220,
220,
1303,
16926,
46,
25,
5409,
543,
3599,
966,
198,
220,
220,
220,
24061,
62,
38690,
62,
4122,
0,
7,
198,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
32,
11,
198,
220,
220,
220,
220,
220,
220,
220,
376,
11,
198,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
34453,
11,
198,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
65,
11,
2746,
13,
66,
11,
2746,
13,
84,
521,
11,
2746,
13,
84,
2100,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
1303,
50200,
2604,
198,
220,
220,
220,
611,
15942,
577,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
44872,
7203,
632,
77,
220,
220,
220,
220,
220,
37712,
38764,
220,
220,
220,
220,
220,
220,
220,
20446,
38764,
220,
220,
220,
11460,
4806,
20446,
4806,
471,
33,
358,
4806,
59,
77,
4943,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
1388,
9052,
198,
220,
220,
220,
1303,
4574,
0,
7,
55,
11,
4866,
7,
19849,
13,
34453,
4008,
628,
220,
220,
220,
981,
299,
2676,
1279,
399,
62,
2043,
1137,
62,
22921,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
314,
13,
5178,
290,
5766,
17321,
4482,
198,
220,
220,
220,
220,
220,
220,
220,
24061,
62,
3605,
1122,
0,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
32,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
34453,
13,
87,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
34453,
13,
82,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
34453,
13,
86,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
34453,
13,
89,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
84,
521,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7377,
116,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
376,
198,
220,
220,
220,
220,
220,
220,
220,
1267,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
2873,
13,
3082,
1133,
290,
1011,
2239,
198,
220,
220,
220,
220,
220,
220,
220,
24061,
62,
19545,
62,
2676,
378,
0,
7,
19849,
11,
376,
8,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
6711,
13,
4897,
12,
19934,
1343,
3359,
2604,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
24061,
29598,
82,
198,
220,
220,
220,
220,
220,
220,
220,
374,
65,
796,
2746,
13,
32,
1635,
2746,
13,
34453,
13,
87,
532,
2746,
13,
65,
198,
220,
220,
220,
220,
220,
220,
220,
48321,
796,
1629,
62,
76,
377,
62,
33,
7,
19849,
13,
32,
11,
2746,
13,
34453,
13,
88,
8,
1343,
2746,
13,
34453,
13,
82,
532,
2746,
13,
66,
198,
220,
220,
220,
220,
220,
220,
220,
599,
87,
15577,
0,
32590,
16,
13,
15,
11,
48321,
11,
2746,
13,
84,
521,
11,
2746,
13,
34453,
13,
89,
8,
198,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
7422,
796,
2746,
13,
34453,
13,
87,
58,
19849,
13,
84,
521,
60,
1343,
2746,
13,
34453,
13,
86,
532,
2746,
13,
84,
2100,
628,
220,
220,
220,
220,
220,
220,
220,
26181,
62,
1050,
4402,
796,
16605,
7,
19849,
13,
34453,
13,
87,
11,
2746,
13,
66,
8,
198,
220,
220,
220,
220,
220,
220,
220,
26181,
62,
646,
282,
796,
16605,
7,
19849,
13,
65,
11,
2746,
13,
34453,
13,
88,
8,
532,
16605,
7,
19849,
13,
84,
2100,
11,
2746,
13,
34453,
13,
89,
8,
628,
220,
220,
220,
220,
220,
220,
220,
299,
2676,
15853,
352,
628,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
2198,
12225,
34054,
198,
220,
220,
220,
220,
220,
220,
220,
304,
862,
62,
79,
796,
357,
27237,
7,
26145,
4008,
1220,
357,
16,
13,
15,
1343,
2593,
7,
19849,
13,
65,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
304,
862,
62,
67,
796,
357,
27237,
7,
6015,
4008,
1220,
357,
16,
13,
15,
1343,
2593,
7,
19849,
13,
66,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
304,
862,
62,
84,
796,
357,
27237,
7,
622,
4008,
1220,
357,
16,
13,
15,
1343,
2593,
7,
19849,
13,
84,
2100,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
304,
862,
62,
70,
796,
2352,
7,
26801,
62,
1050,
4402,
532,
26181,
62,
646,
282,
8,
1220,
357,
16,
13,
15,
1343,
2352,
7,
26801,
62,
1050,
4402,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
611,
15942,
577,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
220,
220,
220,
220,
3601,
7203,
59,
83,
25386,
62,
79,
2625,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
220,
220,
220,
220,
3601,
7,
31,
82,
37435,
7203,
4,
24,
13,
17,
68,
1600,
304,
862,
62,
79,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
220,
220,
220,
220,
3601,
7203,
59,
83,
25386,
62,
67,
2625,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
220,
220,
220,
220,
3601,
7,
31,
82,
37435,
7203,
4,
24,
13,
17,
68,
1600,
304,
862,
62,
67,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
220,
220,
220,
220,
3601,
7203,
59,
83,
25386,
62,
84,
2625,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
220,
220,
220,
220,
3601,
7,
31,
82,
37435,
7203,
4,
24,
13,
17,
68,
1600,
304,
862,
62,
84,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
220,
220,
220,
220,
3601,
7203,
59,
83,
25386,
62,
70,
2625,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
220,
220,
220,
220,
3601,
7,
31,
82,
37435,
7203,
4,
24,
13,
17,
68,
1600,
304,
862,
62,
70,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
220,
220,
220,
220,
44872,
7203,
59,
77,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
886,
198,
220,
220,
220,
220,
220,
220,
220,
611,
15942,
577,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3601,
7,
31,
82,
37435,
7203,
4,
19,
67,
1600,
299,
2676,
4008,
220,
1303,
24415,
954,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3601,
7,
31,
82,
37435,
7203,
4,
10,
1507,
13,
22,
68,
1600,
26181,
62,
1050,
4402,
4008,
220,
1303,
43750,
9432,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3601,
7,
31,
82,
37435,
7203,
4,
10,
1433,
13,
22,
68,
1600,
26181,
62,
646,
282,
4008,
220,
1303,
10668,
9432,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3601,
7,
31,
82,
37435,
7203,
4,
940,
13,
17,
68,
1600,
2593,
7,
26145,
11,
4806,
22305,
220,
1303,
43750,
1167,
30412,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3601,
7,
31,
82,
37435,
7203,
4,
24,
13,
17,
68,
1600,
2593,
7,
6015,
11,
4806,
22305,
220,
1303,
10668,
1167,
30412,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3601,
7,
31,
82,
37435,
7203,
4,
24,
13,
17,
68,
1600,
2593,
7,
622,
11,
4806,
22305,
220,
1303,
6727,
5421,
1167,
30412,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3601,
7,
31,
82,
37435,
7203,
4,
24,
13,
17,
68,
1600,
2352,
7,
26801,
62,
1050,
4402,
532,
26181,
62,
646,
282,
8,
1220,
357,
19849,
13,
48005,
945,
1343,
2546,
7,
19849,
13,
84,
521,
11,
352,
35514,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3601,
7203,
59,
77,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
886,
628,
220,
220,
220,
220,
220,
220,
220,
611,
357,
25386,
62,
79,
1279,
284,
75,
8,
11405,
357,
25386,
62,
84,
1279,
284,
75,
8,
11405,
357,
25386,
62,
67,
1279,
284,
75,
8,
11405,
357,
25386,
62,
70,
1279,
284,
75,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
13376,
796,
1058,
27871,
4402,
198,
220,
220,
220,
220,
220,
220,
220,
886,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
4574,
0,
7,
55,
11,
4866,
7,
19849,
13,
34453,
4008,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
2198,
3722,
198,
220,
220,
220,
220,
220,
220,
220,
611,
2746,
13,
13376,
6624,
1058,
27871,
4402,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
15942,
577,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
44872,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
44872,
7203,
27871,
4402,
4610,
1043,
19570,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1441,
2746,
13,
13376,
198,
220,
220,
220,
220,
220,
220,
220,
886,
628,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
220,
198,
220,
220,
220,
1441,
2746,
13,
13376,
198,
220,
220,
220,
220,
198,
437,
628,
198,
37811,
198,
220,
220,
220,
24061,
62,
38690,
62,
4122,
0,
198,
220,
220,
220,
3082,
1133,
257,
3599,
966,
198,
198,
2,
20559,
2886,
198,
12,
63,
19849,
3712,
17633,
63,
198,
12,
63,
37,
3712,
41384,
1634,
63,
25,
609,
4316,
2584,
5766,
286,
317,
9,
32,
3256,
810,
317,
318,
262,
32315,
17593,
198,
220,
220,
220,
286,
262,
23989,
1917,
13,
198,
37811,
198,
8818,
24061,
62,
38690,
62,
4122,
0,
7,
198,
220,
220,
220,
317,
3712,
23839,
46912,
90,
51,
85,
5512,
198,
220,
220,
220,
376,
3712,
41384,
1634,
90,
51,
85,
5512,
198,
220,
220,
220,
7377,
249,
3712,
51,
377,
541,
13,
23828,
282,
36248,
12727,
90,
51,
85,
5512,
198,
220,
220,
220,
275,
3712,
13290,
1384,
38469,
90,
51,
85,
5512,
198,
220,
220,
220,
269,
3712,
13290,
1384,
38469,
90,
51,
85,
5512,
198,
220,
220,
220,
334,
521,
3712,
13290,
1384,
38469,
90,
40533,
5512,
198,
220,
220,
220,
334,
2100,
3712,
13290,
1384,
38469,
90,
51,
85,
92,
198,
220,
220,
220,
1267,
810,
90,
51,
85,
27,
25,
15633,
11,
16953,
27,
25,
46541,
92,
628,
220,
220,
220,
357,
76,
11,
299,
8,
796,
2546,
7,
32,
8,
198,
220,
220,
220,
279,
796,
2546,
7,
84,
521,
11,
352,
8,
628,
220,
220,
220,
9529,
82,
796,
532,
362,
1635,
275,
198,
220,
220,
220,
334,
62,
796,
1976,
27498,
7,
77,
8,
198,
220,
220,
220,
599,
87,
15577,
0,
7,
16,
13,
15,
11,
334,
62,
11,
334,
521,
11,
334,
2100,
8,
198,
220,
220,
220,
9529,
82,
15853,
317,
9,
334,
62,
628,
220,
220,
220,
1303,
4770,
25609,
855,
2,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
220,
220,
314,
13,
4294,
303,
734,
1195,
12016,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
4770,
25609,
855,
2,
628,
220,
220,
220,
1303,
3082,
1133,
2124,
15,
198,
220,
220,
220,
410,
796,
376,
3467,
9529,
82,
628,
220,
220,
220,
4866,
0,
7,
138,
249,
13,
87,
11,
532,
15,
13,
20,
1635,
1629,
62,
76,
377,
62,
33,
7,
32,
11,
410,
4008,
198,
220,
220,
220,
599,
87,
15577,
0,
7,
15,
13,
20,
11,
7377,
249,
13,
87,
11,
334,
521,
11,
334,
2100,
8,
628,
220,
220,
220,
1303,
3082,
1133,
266,
15,
198,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
287,
352,
25,
79,
198,
220,
220,
220,
220,
220,
220,
220,
474,
796,
334,
521,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
249,
13,
86,
58,
72,
60,
796,
334,
2100,
58,
72,
60,
532,
7377,
249,
13,
87,
58,
73,
60,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
3082,
1133,
331,
15,
198,
220,
220,
220,
4866,
0,
7,
138,
249,
13,
88,
11,
376,
3467,
357,
32,
9,
66,
4008,
628,
220,
220,
220,
1303,
3082,
1133,
264,
15,
198,
220,
220,
220,
4866,
0,
7,
138,
249,
13,
82,
11,
657,
13,
20,
1635,
357,
2953,
62,
76,
377,
62,
33,
7,
32,
11,
7377,
249,
13,
88,
8,
532,
269,
4008,
628,
220,
220,
220,
1303,
3082,
1133,
1976,
15,
198,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
287,
352,
25,
79,
198,
220,
220,
220,
220,
220,
220,
220,
474,
796,
334,
521,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
249,
13,
89,
58,
72,
60,
796,
532,
7377,
249,
13,
82,
58,
73,
60,
198,
220,
220,
220,
886,
628,
198,
220,
220,
220,
1303,
4770,
25609,
855,
2,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
220,
220,
2873,
13,
3082,
1133,
17137,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
4770,
25609,
855,
2,
628,
220,
220,
220,
288,
79,
796,
6632,
7,
51,
85,
8,
198,
220,
220,
220,
49427,
796,
6632,
7,
51,
85,
8,
628,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
287,
352,
25,
77,
198,
220,
220,
220,
220,
220,
220,
220,
45218,
796,
532,
16,
13,
20,
1635,
7377,
249,
13,
87,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
611,
45218,
1875,
288,
79,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
288,
79,
796,
45218,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
287,
352,
25,
79,
198,
220,
220,
220,
220,
220,
220,
220,
45218,
796,
532,
16,
13,
20,
1635,
7377,
249,
13,
86,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
611,
45218,
1875,
288,
79,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
288,
79,
796,
45218,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
198,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
287,
352,
25,
77,
198,
220,
220,
220,
220,
220,
220,
220,
45218,
796,
532,
16,
13,
20,
1635,
7377,
249,
13,
82,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
611,
45218,
1875,
49427,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
49427,
796,
45218,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
287,
352,
25,
79,
198,
220,
220,
220,
220,
220,
220,
220,
45218,
796,
532,
16,
13,
20,
1635,
7377,
249,
13,
89,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
611,
45218,
1875,
49427,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
49427,
796,
45218,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
45218,
796,
16605,
7,
138,
249,
13,
87,
1343,
288,
79,
11,
7377,
249,
13,
82,
1343,
49427,
8,
1343,
16605,
7,
138,
249,
13,
86,
1343,
288,
79,
11,
7377,
249,
13,
89,
1343,
49427,
8,
628,
220,
220,
220,
288,
79,
15853,
657,
13,
20,
1635,
45218,
1220,
357,
16345,
7,
138,
249,
13,
82,
1343,
49427,
8,
1343,
2160,
7,
138,
249,
13,
89,
1343,
49427,
4008,
198,
220,
220,
220,
49427,
15853,
657,
13,
20,
1635,
45218,
1220,
357,
16345,
7,
138,
249,
13,
87,
1343,
288,
79,
8,
1343,
2160,
7,
138,
249,
13,
86,
1343,
288,
79,
4008,
628,
220,
220,
220,
1303,
4770,
25609,
855,
2,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
220,
220,
6711,
13,
27967,
17137,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
4770,
25609,
855,
2,
628,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
287,
352,
25,
77,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
249,
13,
87,
58,
72,
60,
15853,
288,
79,
220,
220,
220,
220,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
287,
352,
25,
77,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
249,
13,
82,
58,
72,
60,
15853,
49427,
220,
220,
220,
220,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
287,
352,
25,
79,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
249,
13,
86,
58,
72,
60,
15853,
288,
79,
220,
220,
220,
220,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
287,
352,
25,
79,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
249,
13,
89,
58,
72,
60,
15853,
49427,
220,
220,
220,
220,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
24429,
198,
220,
220,
220,
1441,
7377,
249,
198,
437,
198,
198,
8818,
24061,
62,
19545,
62,
2676,
378,
0,
7,
19849,
3712,
17633,
11,
376,
3712,
41384,
1634,
8,
198,
220,
220,
220,
357,
87,
11,
331,
11,
264,
11,
266,
11,
1976,
8,
796,
357,
19849,
13,
34453,
13,
87,
11,
2746,
13,
34453,
13,
88,
11,
2746,
13,
34453,
13,
82,
11,
2746,
13,
34453,
13,
86,
11,
2746,
13,
34453,
13,
89,
8,
198,
220,
220,
220,
357,
76,
11,
299,
11,
279,
8,
796,
2746,
13,
77,
1102,
2536,
11,
2746,
13,
48005,
945,
11,
2546,
7,
19849,
13,
84,
521,
11,
352,
8,
628,
220,
220,
220,
288,
62,
2001,
796,
4866,
7,
19849,
13,
34453,
8,
198,
220,
220,
220,
288,
62,
535,
796,
4866,
7,
19849,
13,
34453,
8,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
24061,
29598,
82,
198,
220,
220,
220,
18919,
796,
357,
198,
220,
220,
220,
220,
220,
220,
220,
357,
26518,
7,
87,
11,
264,
8,
1343,
16605,
7,
86,
11,
1976,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
1220,
357,
77,
1343,
279,
8,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
374,
65,
796,
357,
19849,
13,
32,
1635,
2124,
8,
532,
2746,
13,
65,
198,
220,
220,
220,
48321,
796,
1629,
62,
76,
377,
62,
33,
7,
19849,
13,
32,
11,
331,
8,
1343,
264,
532,
2746,
13,
66,
198,
220,
220,
220,
599,
87,
15577,
0,
32590,
16,
13,
15,
11,
48321,
11,
2746,
13,
84,
521,
11,
2746,
13,
34453,
13,
89,
8,
628,
220,
220,
220,
7422,
796,
2124,
58,
19849,
13,
84,
521,
60,
1343,
266,
532,
2746,
13,
84,
2100,
198,
220,
220,
220,
374,
34223,
796,
2124,
764,
9,
264,
198,
220,
220,
220,
374,
86,
89,
796,
266,
764,
9,
1976,
628,
220,
220,
220,
7377,
116,
796,
2124,
24457,
264,
198,
220,
220,
220,
4296,
62,
1169,
8326,
0,
7,
138,
116,
11,
2124,
11,
264,
11,
1976,
11,
266,
11,
2746,
13,
84,
521,
8,
628,
220,
220,
220,
1303,
24061,
41568,
198,
220,
220,
220,
8494,
62,
3605,
1122,
0,
7,
198,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
32,
11,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
116,
11,
198,
220,
220,
220,
220,
220,
220,
220,
376,
11,
198,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
34453,
11,
198,
220,
220,
220,
220,
220,
220,
220,
288,
62,
2001,
11,
198,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
84,
521,
11,
198,
220,
220,
220,
220,
220,
220,
220,
532,
26145,
11,
198,
220,
220,
220,
220,
220,
220,
220,
532,
6015,
11,
198,
220,
220,
220,
220,
220,
220,
220,
532,
622,
11,
198,
220,
220,
220,
220,
220,
220,
220,
532,
81,
34223,
11,
198,
220,
220,
220,
220,
220,
220,
220,
532,
31653,
89,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
1303,
24061,
2239,
4129,
198,
220,
220,
220,
357,
17394,
62,
8957,
11,
26367,
62,
6814,
8,
796,
24061,
62,
9662,
7857,
7,
19849,
13,
34453,
11,
288,
62,
2001,
8,
628,
220,
220,
220,
1303,
4296,
4318,
414,
11507,
198,
220,
220,
220,
18919,
62,
2001,
796,
357,
198,
220,
220,
220,
220,
220,
220,
220,
357,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
16605,
7,
87,
1343,
26367,
62,
8957,
1635,
288,
62,
2001,
13,
87,
11,
264,
1343,
26367,
62,
6814,
1635,
288,
62,
2001,
13,
82,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1343,
16605,
7,
86,
1343,
26367,
62,
8957,
1635,
288,
62,
2001,
13,
86,
11,
1976,
1343,
26367,
62,
6814,
1635,
288,
62,
2001,
13,
89,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1267,
1220,
357,
77,
1343,
279,
8,
198,
220,
220,
220,
1267,
220,
628,
220,
220,
220,
18074,
225,
796,
29405,
19510,
34703,
62,
2001,
1220,
18919,
8,
61,
18,
11,
838,
13,
15,
61,
12,
1065,
11,
352,
13,
15,
532,
838,
13,
15,
61,
12,
1065,
8,
220,
1303,
537,
13322,
329,
29052,
10159,
198,
220,
220,
220,
1303,
24061,
3376,
273,
198,
220,
220,
220,
8494,
62,
3605,
1122,
0,
7,
198,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
32,
11,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
116,
11,
198,
220,
220,
220,
220,
220,
220,
220,
376,
11,
198,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
34453,
11,
198,
220,
220,
220,
220,
220,
220,
220,
288,
62,
535,
11,
198,
220,
220,
220,
220,
220,
220,
220,
2746,
13,
84,
521,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1976,
27498,
7,
76,
828,
198,
220,
220,
220,
220,
220,
220,
220,
1976,
27498,
7,
77,
828,
198,
220,
220,
220,
220,
220,
220,
220,
1976,
27498,
7,
79,
828,
198,
220,
220,
220,
220,
220,
220,
220,
18074,
225,
9,
34703,
9,
1952,
7,
77,
8,
532,
288,
62,
2001,
13,
87,
764,
9,
288,
62,
2001,
13,
82,
11,
198,
220,
220,
220,
220,
220,
220,
220,
18074,
225,
9,
34703,
9,
1952,
7,
79,
8,
532,
288,
62,
2001,
13,
86,
764,
9,
288,
62,
2001,
13,
89,
198,
220,
220,
220,
1267,
628,
220,
220,
220,
1303,
2457,
2239,
2546,
198,
220,
220,
220,
288,
796,
288,
62,
2001,
1343,
288,
62,
535,
198,
220,
220,
220,
357,
17394,
62,
79,
11,
26367,
62,
67,
8,
796,
24061,
62,
9662,
7857,
7,
19849,
13,
34453,
11,
288,
11,
21151,
28,
15,
13,
24214,
20,
8,
628,
220,
220,
220,
1303,
1011,
2239,
198,
220,
220,
220,
2746,
13,
34453,
13,
87,
15853,
26367,
62,
79,
1635,
288,
13,
87,
198,
220,
220,
220,
2746,
13,
34453,
13,
88,
15853,
26367,
62,
67,
1635,
288,
13,
88,
198,
220,
220,
220,
2746,
13,
34453,
13,
82,
15853,
26367,
62,
67,
1635,
288,
13,
82,
198,
220,
220,
220,
2746,
13,
34453,
13,
86,
15853,
26367,
62,
79,
1635,
288,
13,
86,
198,
220,
220,
220,
2746,
13,
34453,
13,
89,
15853,
26367,
62,
67,
1635,
288,
13,
89,
628,
220,
220,
220,
1441,
2746,
13,
34453,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
18975,
62,
354,
4316,
2584,
198,
220,
220,
220,
3082,
1133,
609,
4316,
2584,
5766,
1634,
286,
317,
9,
32,
6,
198,
37811,
198,
8818,
18975,
62,
354,
4316,
2584,
7,
32,
3712,
23839,
46912,
90,
51,
30072,
810,
1391,
51,
27,
25,
15633,
92,
628,
220,
220,
220,
376,
796,
609,
4316,
2584,
13,
354,
4316,
2584,
7,
32,
11,
3392,
7,
32,
13,
77,
4008,
198,
220,
220,
220,
1441,
376,
198,
198,
437,
628,
198,
37811,
198,
220,
220,
220,
24061,
62,
3605,
1122,
0,
198,
220,
220,
220,
5178,
290,
5766,
1096,
262,
17321,
1080,
11,
1262,
262,
3487,
27490,
13,
198,
37811,
198,
8818,
24061,
62,
3605,
1122,
0,
7,
198,
220,
220,
220,
317,
3712,
23839,
46912,
90,
38586,
5512,
198,
220,
220,
220,
2124,
3712,
23839,
38469,
90,
46047,
5512,
198,
220,
220,
220,
264,
3712,
23839,
38469,
90,
33758,
5512,
198,
220,
220,
220,
266,
3712,
23839,
38469,
90,
5080,
5512,
198,
220,
220,
220,
1976,
3712,
23839,
38469,
90,
51,
89,
5512,
198,
220,
220,
220,
334,
521,
3712,
23839,
38469,
90,
40533,
5512,
198,
220,
220,
220,
7377,
116,
3712,
23839,
38469,
90,
51,
5512,
198,
220,
220,
220,
376,
3712,
41384,
1634,
90,
38586,
92,
198,
220,
220,
220,
1267,
810,
1391,
38586,
27,
25,
15633,
11,
309,
87,
27,
25,
15633,
11,
13146,
27,
25,
15633,
11,
1815,
27,
25,
15633,
11,
309,
89,
27,
25,
15633,
11,
16953,
27,
25,
46541,
11,
309,
27,
25,
15633,
92,
628,
220,
220,
220,
1303,
3082,
1133,
7377,
246,
796,
357,
55,
36796,
12,
16,
92,
311,
1343,
370,
36796,
12,
16,
92,
1168,
8,
36796,
12,
16,
92,
198,
220,
220,
220,
7377,
116,
796,
2124,
24457,
264,
198,
220,
220,
220,
329,
1312,
287,
352,
25,
7857,
7,
84,
521,
11,
352,
8,
198,
220,
220,
220,
220,
220,
220,
220,
474,
796,
334,
521,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
116,
58,
73,
60,
796,
352,
13,
15,
1220,
357,
82,
58,
73,
60,
1220,
2124,
58,
73,
60,
1343,
1976,
58,
72,
60,
1220,
266,
58,
72,
12962,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
5178,
262,
3487,
27490,
17593,
290,
24061,
663,
5766,
1634,
198,
220,
220,
220,
609,
4316,
2584,
13,
354,
4316,
2584,
0,
7,
32,
11,
7377,
116,
11,
376,
8,
628,
220,
220,
220,
1441,
7377,
116,
198,
437,
628,
198,
37811,
198,
220,
220,
220,
8494,
62,
3605,
1122,
198,
220,
220,
220,
4294,
303,
17321,
1080,
351,
262,
1813,
826,
12,
4993,
1735,
13,
198,
220,
220,
220,
3827,
8933,
274,
262,
5128,
288,
198,
37811,
198,
8818,
8494,
62,
3605,
1122,
0,
7,
198,
220,
220,
220,
317,
3712,
23839,
46912,
90,
38586,
5512,
198,
220,
220,
220,
7377,
116,
3712,
23839,
38469,
90,
51,
16,
5512,
198,
220,
220,
220,
376,
3712,
41384,
1634,
90,
38586,
5512,
198,
220,
220,
220,
7377,
249,
3712,
23828,
282,
36248,
12727,
11,
198,
220,
220,
220,
288,
3712,
23828,
282,
36248,
12727,
11,
198,
220,
220,
220,
334,
521,
3712,
23839,
38469,
90,
40533,
5512,
198,
220,
220,
220,
7377,
122,
62,
65,
3712,
23839,
38469,
90,
51,
17,
5512,
198,
220,
220,
220,
7377,
122,
62,
66,
3712,
23839,
38469,
90,
51,
18,
5512,
198,
220,
220,
220,
7377,
122,
62,
84,
3712,
23839,
38469,
90,
51,
19,
5512,
198,
220,
220,
220,
7377,
122,
62,
34223,
3712,
23839,
38469,
90,
51,
20,
5512,
198,
220,
220,
220,
7377,
122,
62,
86,
89,
3712,
23839,
38469,
90,
51,
21,
5512,
198,
8,
810,
1391,
38586,
27,
25,
15633,
11,
309,
16,
27,
25,
15633,
11,
309,
17,
27,
25,
15633,
11,
309,
18,
27,
25,
15633,
11,
309,
19,
27,
25,
15633,
11,
309,
20,
27,
25,
15633,
11,
309,
21,
27,
25,
15633,
11,
16953,
27,
25,
46541,
92,
628,
220,
220,
220,
7377,
122,
62,
22065,
796,
7377,
122,
62,
66,
532,
357,
138,
122,
62,
34223,
24457,
7377,
249,
13,
87,
8,
198,
220,
220,
220,
7377,
122,
62,
22065,
58,
84,
521,
60,
15853,
357,
138,
122,
62,
86,
89,
532,
357,
138,
249,
13,
89,
764,
9,
7377,
122,
62,
84,
4008,
24457,
7377,
249,
13,
86,
628,
220,
220,
220,
288,
13,
88,
796,
376,
3467,
357,
138,
122,
62,
65,
1343,
317,
1635,
357,
138,
116,
764,
9,
7377,
122,
62,
22065,
4008,
628,
220,
220,
220,
288,
13,
87,
796,
7377,
116,
764,
9,
357,
2953,
62,
76,
377,
62,
33,
7,
32,
11,
288,
13,
88,
8,
532,
7377,
122,
62,
22065,
8,
628,
220,
220,
220,
288,
13,
89,
796,
357,
138,
249,
13,
89,
764,
9,
13841,
138,
122,
62,
84,
1343,
288,
13,
87,
58,
84,
521,
12962,
1343,
7377,
122,
62,
86,
89,
8,
24457,
7377,
249,
13,
86,
198,
220,
220,
220,
288,
13,
82,
796,
357,
138,
122,
62,
34223,
532,
7377,
249,
13,
82,
764,
9,
288,
13,
87,
8,
24457,
7377,
249,
13,
87,
198,
220,
220,
220,
288,
13,
86,
796,
357,
138,
122,
62,
86,
89,
532,
7377,
249,
13,
86,
764,
9,
288,
13,
89,
8,
24457,
7377,
249,
13,
89,
628,
220,
220,
220,
1303,
1303,
2198,
611,
1080,
318,
16019,
9380,
198,
220,
220,
220,
1303,
374,
65,
796,
7377,
122,
62,
65,
532,
317,
9,
67,
13,
87,
198,
220,
220,
220,
1303,
48321,
796,
7377,
122,
62,
66,
532,
357,
32,
6,
9,
67,
13,
88,
1343,
288,
13,
82,
8,
198,
220,
220,
220,
1303,
48321,
58,
84,
521,
60,
15853,
288,
13,
89,
198,
220,
220,
220,
1303,
7422,
796,
7377,
122,
62,
84,
532,
357,
67,
13,
87,
58,
84,
521,
60,
1343,
288,
13,
86,
8,
198,
220,
220,
220,
1303,
374,
34223,
796,
7377,
122,
62,
34223,
532,
357,
138,
249,
13,
82,
764,
9,
288,
13,
87,
1343,
7377,
249,
13,
87,
764,
9,
288,
13,
82,
8,
198,
220,
220,
220,
1303,
374,
86,
89,
796,
7377,
122,
62,
86,
89,
532,
357,
138,
249,
13,
89,
764,
9,
288,
13,
86,
1343,
7377,
249,
13,
86,
764,
9,
288,
13,
89,
8,
628,
220,
220,
220,
1303,
44872,
7203,
4965,
312,
723,
82,
59,
83,
7,
11265,
37430,
82,
8,
4943,
198,
220,
220,
220,
1303,
44872,
7203,
15886,
26145,
15886,
220,
220,
3467,
83,
1600,
2488,
82,
37435,
7203,
7225,
21,
68,
1600,
5415,
7,
8937,
12195,
26145,
35514,
198,
220,
220,
220,
1303,
44872,
7203,
15886,
6015,
15886,
220,
220,
3467,
83,
1600,
2488,
82,
37435,
7203,
7225,
21,
68,
1600,
5415,
7,
8937,
12195,
6015,
35514,
198,
220,
220,
220,
1303,
44872,
7203,
15886,
622,
15886,
220,
220,
3467,
83,
1600,
2488,
82,
37435,
7203,
7225,
21,
68,
1600,
5415,
7,
8937,
12195,
622,
35514,
198,
220,
220,
220,
1303,
44872,
7203,
15886,
81,
34223,
15886,
220,
3467,
83,
1600,
2488,
82,
37435,
7203,
7225,
21,
68,
1600,
5415,
7,
8937,
12195,
81,
34223,
35514,
198,
220,
220,
220,
1303,
44872,
7203,
15886,
31653,
89,
15886,
220,
3467,
83,
1600,
2488,
82,
37435,
7203,
7225,
21,
68,
1600,
5415,
7,
8937,
12195,
31653,
89,
35514,
198,
220,
220,
220,
1303,
44872,
3419,
198,
220,
220,
220,
1441,
288,
198,
437,
628,
198,
8818,
24061,
62,
9662,
7857,
7,
198,
220,
220,
220,
27765,
3712,
23839,
38469,
90,
51,
5512,
665,
3712,
23839,
38469,
90,
51,
5512,
40379,
3712,
23839,
38469,
90,
51,
5512,
256,
89,
3712,
23839,
38469,
90,
51,
5512,
198,
220,
220,
220,
44332,
3712,
23839,
38469,
90,
51,
5512,
43756,
3712,
23839,
38469,
90,
51,
5512,
288,
82,
3712,
23839,
38469,
90,
51,
5512,
288,
89,
3712,
23839,
38469,
90,
51,
19629,
198,
220,
220,
220,
21151,
28,
16,
13,
15,
198,
8,
810,
309,
27,
25,
15633,
628,
220,
220,
220,
299,
796,
2546,
7,
17602,
11,
352,
8,
198,
220,
220,
220,
279,
796,
2546,
7,
4246,
11,
352,
8,
198,
220,
220,
220,
299,
6624,
2546,
7,
912,
11,
352,
8,
8614,
3714,
7,
29271,
3004,
44,
1042,
963,
7203,
83,
13,
82,
318,
2642,
2546,
48774,
198,
220,
220,
220,
279,
6624,
2546,
7,
22877,
11,
352,
8,
8614,
3714,
7,
29271,
3004,
44,
1042,
963,
7203,
83,
13,
89,
318,
2642,
2546,
48774,
198,
220,
220,
220,
220,
198,
220,
220,
220,
299,
6624,
2546,
7,
34350,
11,
352,
8,
8614,
3714,
7,
29271,
3004,
44,
1042,
963,
7203,
67,
13,
87,
318,
2642,
2546,
48774,
198,
220,
220,
220,
299,
6624,
2546,
7,
9310,
11,
352,
8,
8614,
3714,
7,
29271,
3004,
44,
1042,
963,
7203,
67,
13,
82,
318,
2642,
2546,
48774,
198,
220,
220,
220,
279,
6624,
2546,
7,
67,
86,
11,
352,
8,
8614,
3714,
7,
29271,
3004,
44,
1042,
963,
7203,
67,
13,
86,
318,
2642,
2546,
48774,
198,
220,
220,
220,
279,
6624,
2546,
7,
67,
89,
11,
352,
8,
8614,
3714,
7,
29271,
3004,
44,
1042,
963,
7203,
67,
13,
89,
318,
2642,
2546,
48774,
198,
220,
220,
220,
220,
198,
220,
220,
220,
2471,
11,
512,
796,
532,
16,
13,
15,
11,
532,
16,
13,
15,
198,
220,
220,
220,
220,
198,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
287,
352,
25,
77,
198,
220,
220,
220,
220,
220,
220,
220,
611,
44332,
58,
72,
60,
1279,
657,
13,
15,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
357,
17602,
58,
72,
60,
1220,
44332,
58,
72,
12962,
1875,
2471,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2471,
796,
357,
17602,
58,
72,
60,
1220,
44332,
58,
72,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
220,
198,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
287,
352,
25,
77,
198,
220,
220,
220,
220,
220,
220,
220,
611,
288,
82,
58,
72,
60,
1279,
657,
13,
15,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
357,
912,
58,
72,
60,
1220,
288,
82,
58,
72,
12962,
1875,
512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
512,
796,
357,
912,
58,
72,
60,
1220,
288,
82,
58,
72,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
220,
198,
220,
220,
220,
2488,
259,
65,
3733,
329,
474,
287,
352,
25,
79,
198,
220,
220,
220,
220,
220,
220,
220,
611,
43756,
58,
73,
60,
1279,
657,
13,
15,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
357,
4246,
58,
73,
60,
1220,
43756,
58,
73,
12962,
1875,
2471,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2471,
796,
357,
4246,
58,
73,
60,
1220,
43756,
58,
73,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
220,
198,
220,
220,
220,
2488,
259,
65,
3733,
329,
474,
287,
352,
25,
79,
198,
220,
220,
220,
220,
220,
220,
220,
611,
288,
89,
58,
73,
60,
1279,
657,
13,
15,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
357,
22877,
58,
73,
60,
1220,
288,
89,
58,
73,
12962,
1875,
512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
512,
796,
357,
22877,
58,
73,
60,
1220,
288,
89,
58,
73,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
220,
198,
220,
220,
220,
2471,
796,
532,
21151,
1635,
2471,
198,
220,
220,
220,
512,
796,
532,
21151,
1635,
512,
628,
220,
220,
220,
1303,
44872,
7203,
59,
83,
7,
499,
11,
512,
8,
796,
33172,
357,
499,
11,
512,
4008,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1441,
357,
499,
11,
512,
8,
198,
198,
437,
198,
198,
8818,
24061,
62,
9662,
7857,
7,
83,
3712,
23828,
282,
36248,
12727,
90,
51,
5512,
288,
3712,
23828,
282,
36248,
12727,
90,
51,
19629,
21151,
28,
16,
13,
15,
8,
810,
309,
27,
25,
15633,
198,
220,
220,
220,
357,
499,
11,
512,
8,
796,
24061,
62,
9662,
7857,
7,
83,
13,
87,
11,
256,
13,
86,
11,
256,
13,
82,
11,
256,
13,
89,
11,
288,
13,
87,
11,
288,
13,
86,
11,
288,
13,
82,
11,
288,
13,
89,
11,
21151,
28,
67,
696,
8,
198,
220,
220,
220,
1441,
357,
499,
11,
512,
8,
198,
437,
198,
198,
8818,
4296,
62,
1169,
8326,
0,
7,
138,
116,
11,
2124,
11,
264,
11,
1976,
11,
266,
11,
951,
521,
8,
198,
220,
220,
220,
1303,
691,
1444,
422,
1626,
262,
23989,
11,
523,
22303,
547,
10667,
878,
198,
220,
220,
220,
329,
1312,
287,
352,
25,
7857,
7,
4033,
521,
11,
352,
8,
198,
220,
220,
220,
220,
220,
220,
220,
474,
796,
951,
521,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
116,
58,
73,
60,
796,
352,
13,
15,
1220,
357,
82,
58,
73,
60,
1220,
2124,
58,
73,
60,
1343,
1976,
58,
72,
60,
1220,
266,
58,
72,
12962,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
2147,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
599,
87,
15577,
0,
7,
17394,
11,
2124,
11,
331,
62,
521,
11,
331,
62,
2100,
8,
198,
198,
818,
12,
5372,
29964,
286,
2124,
15853,
26367,
1635,
331,
11,
810,
331,
796,
29877,
7,
88,
62,
521,
11,
331,
62,
2100,
8,
198,
198,
2,
20559,
2886,
198,
37811,
198,
8818,
599,
87,
15577,
0,
7,
17394,
3712,
51,
85,
11,
2124,
3712,
23839,
38469,
90,
51,
85,
5512,
331,
62,
521,
3712,
23839,
38469,
90,
40533,
5512,
331,
62,
2100,
3712,
23839,
38469,
90,
51,
85,
30072,
810,
90,
40533,
27,
25,
46541,
11,
309,
85,
27,
25,
15633,
92,
198,
220,
220,
220,
329,
1312,
287,
352,
25,
7857,
7,
88,
62,
521,
11,
352,
8,
198,
220,
220,
220,
220,
220,
220,
220,
474,
796,
331,
62,
521,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
58,
73,
60,
796,
2124,
58,
73,
60,
1343,
26367,
1635,
331,
62,
2100,
58,
72,
60,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
2147,
198,
437,
198
] | 1.872774 | 6,681 |
# # [Utilities (util.jl)](@id util)
# Unlike every other file in the pipeline, this file is not intended to be run directly.
# Instead, include this in other files. These utilities provide an interface to the Planck
# data products, namely
# 1. binning matrix
# 2. beam ``W_{\ell}^{XY} = B_{\ell}^X B_{\ell}^{Y}``
# 3. foreground model cross-spectra
# 4. ``\texttt{plic}`` reference covariance matrix and reference spectra, for comparison plots
using PowerSpectra
using DataFrames, CSV
using DelimitedFiles
using LinearAlgebra
using FITSIO
# ## Planck Binning
# Planck bins the spectra at the very end, and applies an ``\ell (\ell+1)`` relative
# weighting inside the bin. This utility function generates the binning operator
# ``P_{b\ell}`` such that ``C_b = P_{b \ell} C_{\ell}``. It also returns the mean of the
# left and right bin edges, which is what is used when plotting the Planck spectra.
"""
util_planck_binning(binfile; lmax=6143)
Obtain the Planck binning scheme.
### Arguments:
- `binfile::String`: filename of the Planck binning, containing left/right bin edges
### Keywords
- `lmax::Int=6143`: maximum multipole for one dimension of the binning matrix
### Returns:
- `Tuple{Matrix{Float64}, Vector{Float64}`: returns (binning matrix, bin centers)
"""
function util_planck_binning(binfile; lmax=6143)
bin_df = DataFrame(CSV.File(binfile;
header=false, delim=" ", ignorerepeated=true))
lb = (bin_df[:,1] .+ bin_df[:,2]) ./ 2
P = binning_matrix(bin_df[:,1], bin_df[:,2], ℓ -> ℓ*(ℓ+1) / (2π); lmax=lmax)
return P, lb[1:size(P,1)]
end
# ## Planck Beam
# The Planck effective beams are azimuthally-averaged window functions induced by the
# instrumental optics. This utility function reads the Planck beams from the RIMO, which
# are of the form `TT_2_TT`, `TT_2_EE` etc. Conventionally, the Planck spectra are stored
# with the diagonal of the beam-mixing matrix applied.
#
# ```math
# C_{\ell} = W^{-1}_{\ell} \hat{C}_{\ell}
# ```
"""
util_planck_beam_Wl([T::Type=Float64], freq1, split1, freq2, split2, spec1_, spec2_;
lmax=4000, beamdir=nothing)
Returns the Planck beam transfer of [spec1]_to_[spec2], in Wl form.
### Arguments:
- `T::Type=Float64`: optional first parameter specifying numerical type
- `freq1::String`: frequency of first field
- `split1::String`: split of first field (i.e. hm1)
- `freq2::String`: frequency of first field
- `split2::String`: split of second field (i.e. hm2)
- `spec1_`: converted to string, source spectrum like TT
- `spec2_`: converted to string, destination spectrum
### Keywords
- `lmax=4000`: maximum multipole
- `beamdir=nothing`: directory containing beam FITS files. if nothing, will fall back to
the PowerSpectra.jl beam files.
### Returns:
- `SpectralVector`: the beam Wl, indexed 0:lmax
"""
function util_planck_beam_Wl(T::Type, freq1, split1, freq2, split2, spec1_, spec2_;
lmax=4000, beamdir=nothing)
if isnothing(beamdir)
@warn "beam directory not specified. switching to PowerSpectra.jl fallback"
beamdir = PowerSpectra.planck256_beamdir()
end
spec1 = String(spec1_)
spec2 = String(spec2_)
if parse(Int, freq1) > parse(Int, freq2)
freq1, freq2 = freq2, freq1
split1, split2 = split2, split1
end
if (freq1 == freq2) && ((split1 == "hm2") && (split2 == "hm1"))
split1, split2 = split2, split1
end
fname = "Wl_R3.01_plikmask_$(freq1)$(split1)x$(freq2)$(split2).fits"
f = FITS(joinpath(beamdir, "BeamWf_HFI_R3.01", fname))
Wl = convert(Vector{T}, read(f[spec1], "$(spec1)_2_$(spec2)")[:,1])
if lmax < 4000
Wl = Wl[1:lmax+1]
else
Wl = vcat(Wl, last(Wl) * ones(T, lmax - 4000))
end
return SpectralVector(Wl)
end
util_planck_beam_Wl(T::Type, freq1, split1, freq2, split2, spec1; kwargs...) =
util_planck_beam_Wl(T, freq1, split1, freq2, split2, spec1, spec1; kwargs...)
util_planck_beam_Wl(freq1::String, split1, freq2, split2, spec1, spec2; kwargs...) =
util_planck_beam_Wl(Float64, freq1, split1, freq2, split2, spec1, spec2; kwargs...)
# ## Planck Likelihood Specifics
# The Planck likelihood uses a specific choice of spectra and multipole ranges for those
# spectra. We provide some utility functions to retrieve a copy of the spectra order and
# the multipole minimum and maximum for those spectra.
plic_order() = (
(:TT,"100","100"), (:TT,"143","143"), (:TT,"143","217"), (:TT,"217","217"),
(:EE,"100","100"), (:EE,"100","143"), (:EE,"100","217"), (:EE,"143","143"),
(:EE,"143","217"), (:EE,"217","217"),
(:TE,"100","100"), (:TE,"100","143"), (:TE,"100","217"), (:TE,"143","143"),
(:TE,"143","217"), (:TE,"217","217")
)
const plic_ellranges = Dict(
(:TT, "100", "100") => (30, 1197),
(:TT, "143", "143") => (30, 1996),
(:TT, "143", "217") => (30, 2508),
(:TT, "217", "217") => (30, 2508),
(:EE, "100", "100") => (30, 999),
(:EE, "100", "143") => (30, 999),
(:EE, "100", "217") => (505, 999),
(:EE, "143", "143") => (30, 1996),
(:EE, "143", "217") => (505, 1996),
(:EE, "217", "217") => (505, 1996),
(:TE, "100", "100") => (30, 999),
(:TE, "100", "143") => (30, 999),
(:TE, "100", "217") => (505, 999),
(:TE, "143", "143") => (30, 1996),
(:TE, "143", "217") => (505, 1996),
(:TE, "217", "217") => (505, 1996),
(:TT, "100", "143") => (30, 999), # not used
(:TT, "100", "217") => (505, 999), # not used
)
function get_plic_ellrange(spec::Symbol, freq1, freq2)
if spec ∈ (:TE, :ET)
if parse(Float64, freq1) > parse(Float64, freq2)
freq1, freq2 = freq2, freq1
end
return plic_ellranges[:TE, freq1, freq2]
end
return plic_ellranges[spec, freq1, freq2]
end
# ## Signal Spectra
# The covariance matrix calculation and and signal simulations require an assumed signal
# spectra. We use the same foreground spectra as used in the ``\text{plic}`` likelihood.
# This returns dictionaries for signal and theory ``C_{\ell}`` in ``\mu\mathrm{K}``
# between two frequencies. The data is stored in the `plicref` directory in the config.
function signal_and_theory(freq1, freq2, config::Dict)
likelihood_data_dir = joinpath(config["scratch"], "plicref")
th = read_commented_header(joinpath(likelihood_data_dir,"theory_cl.txt"))
fg = read_commented_header(joinpath(likelihood_data_dir,
"base_plikHM_TTTEEE_lowl_lowE_lensing.minimum.plik_foregrounds"))
for (spec, f1, f2) in plic_order()
lmin, lmax = get_plic_ellrange(spec, f1, f2)
const_val = fg[lmax-1,"$(spec)$(f1)X$(f2)"]
## constant foreground level after lmax -- there are fitting artifacts otherwise
fg[(lmax-1):end, "$(spec)$(f1)X$(f2)"] .= const_val
end
## loop over spectra and also fill in the flipped name
freqs = ("100", "143", "217")
specs = ("TT", "TE", "ET", "EE")
for f1 in freqs, f2 in freqs, spec in specs
if "$(spec)$(f1)X$(f2)" ∉ names(fg)
if "$(reverse(spec))$(f2)X$(f1)" ∈ names(fg)
fg[!, "$(spec)$(f1)X$(f2)"] = fg[!, "$(reverse(spec))$(f2)X$(f1)"]
else
fg[!, "$(spec)$(f1)X$(f2)"] = zeros(nrow(fg))
end
end
end
ap(v) = vcat([0., 0.], v)
ell_fac = fg[!, "l"] .* (fg[!, "l"] .+ 1) ./ (2π);
signal_dict = Dict{String,Vector{Float64}}()
theory_dict = Dict{String,Vector{Float64}}()
for XY₀ in specs ## XY₀ is the spectrum to store
f₁, f₂ = parse(Int, freq1), parse(Int, freq2)
if f₁ <= f₂
XY = XY₀
else ## swap what we're looking for, as fg data only has those cross-spectra
XY = XY₀[2] * XY₀[1]
f₁, f₂ = f₂, f₁
end
if XY == "ET"
theory_cl_XY = th[!, "TE"] ./ (th[!, "L"] .* (th[!, "L"] .+ 1) ./ (2π))
else
theory_cl_XY = th[!, XY] ./ (th[!, "L"] .* (th[!, "L"] .+ 1) ./ (2π))
end
fg_cl_XY = fg[!, "$(XY)$(f₁)X$(f₂)"] ./ (fg[!, "l"] .* (fg[!, "l"] .+ 1) ./ (2π))
signal_dict[XY₀] = ap(theory_cl_XY .+ fg_cl_XY[1:2507])
theory_dict[XY₀] = ap(theory_cl_XY)
end
return signal_dict, theory_dict
end
# ## Planck ``\texttt{plic}`` Reference
# In various parts of the pipeline, we want to compare our results to the official 2018
# data release. These routines load them from disk. They're automatically downloaded to the
# `plicref` directory specified in the configuration TOML.
"""
PlanckReferenceCov(plicrefpath::String)
Stores the spectra and covariances of the reference plic analysis.
"""
PlanckReferenceCov
struct PlanckReferenceCov{T}
cov::Array{T,2}
ells::Vector{Int}
cls::Vector{T}
keys::Vector{String}
sub_indices::Vector{Int}
key_index_dict::Dict{String,Int}
end
function PlanckReferenceCov(plicrefpath)
ellspath = joinpath(plicrefpath, "vec_all_spectra.dat")
clpath = joinpath(plicrefpath, "data_extracted.dat")
covpath = joinpath(plicrefpath, "covmat.dat")
keys = ["TT_100x100", "TT_143x143", "TT_143x217", "TT_217x217", "EE_100x100",
"EE_100x143", "EE_100x217", "EE_143x143", "EE_143x217", "EE_217x217", "TE_100x100",
"TE_100x143", "TE_100x217", "TE_143x143", "TE_143x217", "TE_217x217"]
cov = inv(readdlm(covpath))
ells = readdlm(ellspath)[:,1]
cls = readdlm(clpath)[:,2]
subarray_indices = collect(0:(size(cov,1)-2))[findall(diff(ells) .< 0) .+ 1] .+ 1
sub_indices = [1, subarray_indices..., length(cls)+1]
key_ind = 1:length(keys)
key_index_dict = Dict(keys .=> key_ind)
return PlanckReferenceCov{Float64}(cov, ells, cls, keys, sub_indices, key_index_dict)
end
"""
get_subcov(pl::PlanckReferenceCov, spec1, spec2)
Extract the sub-covariance matrix corresponding to spec1 × spec2.
### Arguments:
- `pl::PlanckReferenceCov`: data structure storing reference covmat and spectra
- `spec1::String`: spectrum of form i.e. "TT_100x100"
- `spec2::String`: spectrum of form i.e. "TT_100x100"
### Returns:
- `Matrix{Float64}`: subcovariance matrix
"""
function get_subcov(pl::PlanckReferenceCov, spec1, spec2)
i = pl.key_index_dict[spec1]
j = pl.key_index_dict[spec2]
return pl.cov[
pl.sub_indices[i] : (pl.sub_indices[i + 1] - 1),
pl.sub_indices[j] : (pl.sub_indices[j + 1] - 1),
]
end
"""
extract_spec_and_cov(pl::PlanckReferenceCov, spec1)
Extract the reference ells, cl, errorbar, and sub-covariance block for a spectrum × itself.
### Arguments:
- `pl::PlanckReferenceCov`: data structure storing reference covmat and spectra
- `spec1::String`: spectrum of form i.e. "TT_100x100"
### Returns:
- `(ells, cl, err, this_subcov)`
"""
function extract_spec_and_cov(pl::PlanckReferenceCov, spec1)
i = pl.key_index_dict[spec1]
this_subcov = get_subcov(pl, spec1, spec1)
ells = pl.ells[pl.sub_indices[i]:(pl.sub_indices[i + 1] - 1)]
cl = pl.cls[pl.sub_indices[i]:(pl.sub_indices[i + 1] - 1)]
err = sqrt.(diag(this_subcov))
return ells, cl, err, this_subcov
end
| [
2,
1303,
685,
18274,
2410,
357,
22602,
13,
20362,
15437,
7,
31,
312,
7736,
8,
198,
2,
12101,
790,
584,
2393,
287,
262,
11523,
11,
428,
2393,
318,
407,
5292,
284,
307,
1057,
3264,
13,
198,
2,
5455,
11,
2291,
428,
287,
584,
3696,
13,
2312,
20081,
2148,
281,
7071,
284,
262,
5224,
694,
198,
2,
1366,
3186,
11,
14811,
220,
198,
2,
352,
13,
9874,
768,
17593,
198,
2,
362,
13,
15584,
7559,
54,
23330,
59,
695,
92,
36796,
34278,
92,
796,
347,
23330,
59,
695,
92,
61,
55,
347,
23330,
59,
695,
92,
36796,
56,
92,
15506,
198,
2,
513,
13,
36282,
2746,
3272,
12,
4443,
430,
198,
2,
604,
13,
7559,
59,
5239,
926,
90,
489,
291,
92,
15506,
4941,
44829,
590,
17593,
290,
4941,
5444,
430,
11,
329,
7208,
21528,
628,
198,
3500,
4333,
49738,
430,
198,
3500,
6060,
35439,
11,
44189,
198,
3500,
4216,
320,
863,
25876,
198,
3500,
44800,
2348,
29230,
198,
3500,
376,
29722,
9399,
198,
198,
2,
22492,
5224,
694,
20828,
768,
220,
198,
2,
5224,
694,
41701,
262,
5444,
430,
379,
262,
845,
886,
11,
290,
8991,
281,
7559,
59,
695,
357,
59,
695,
10,
16,
8,
15506,
3585,
220,
198,
2,
3463,
278,
2641,
262,
9874,
13,
770,
10361,
2163,
18616,
262,
9874,
768,
10088,
220,
198,
2,
7559,
47,
23330,
65,
59,
695,
92,
15506,
884,
326,
7559,
34,
62,
65,
796,
350,
23330,
65,
3467,
695,
92,
327,
23330,
59,
695,
92,
15506,
13,
632,
635,
5860,
262,
1612,
286,
262,
220,
198,
2,
1364,
290,
826,
9874,
13015,
11,
543,
318,
644,
318,
973,
618,
29353,
262,
5224,
694,
5444,
430,
13,
198,
198,
37811,
198,
220,
220,
220,
7736,
62,
11578,
694,
62,
8800,
768,
7,
8800,
7753,
26,
300,
9806,
28,
21,
21139,
8,
198,
198,
5944,
3153,
262,
5224,
694,
9874,
768,
7791,
13,
198,
198,
21017,
20559,
2886,
25,
198,
12,
4600,
8800,
7753,
3712,
10100,
63,
25,
29472,
286,
262,
5224,
694,
9874,
768,
11,
7268,
1364,
14,
3506,
9874,
13015,
198,
198,
21017,
7383,
10879,
198,
12,
4600,
75,
9806,
3712,
5317,
28,
21,
21139,
63,
25,
5415,
18540,
2305,
329,
530,
15793,
286,
262,
9874,
768,
17593,
198,
198,
21017,
16409,
25,
220,
198,
12,
4600,
51,
29291,
90,
46912,
90,
43879,
2414,
5512,
20650,
90,
43879,
2414,
92,
63,
25,
5860,
357,
8800,
768,
17593,
11,
9874,
10399,
8,
198,
37811,
198,
8818,
7736,
62,
11578,
694,
62,
8800,
768,
7,
8800,
7753,
26,
300,
9806,
28,
21,
21139,
8,
198,
220,
220,
220,
9874,
62,
7568,
796,
6060,
19778,
7,
7902,
53,
13,
8979,
7,
8800,
7753,
26,
220,
198,
220,
220,
220,
220,
220,
220,
220,
13639,
28,
9562,
11,
46728,
2625,
33172,
8856,
45956,
515,
28,
7942,
4008,
198,
220,
220,
220,
18360,
796,
357,
8800,
62,
7568,
58,
45299,
16,
60,
764,
10,
9874,
62,
7568,
58,
45299,
17,
12962,
24457,
362,
198,
220,
220,
220,
350,
796,
9874,
768,
62,
6759,
8609,
7,
8800,
62,
7568,
58,
45299,
16,
4357,
9874,
62,
7568,
58,
45299,
17,
4357,
2343,
226,
241,
4613,
2343,
226,
241,
9,
7,
158,
226,
241,
10,
16,
8,
1220,
357,
17,
46582,
1776,
300,
9806,
28,
75,
9806,
8,
198,
220,
220,
220,
1441,
350,
11,
18360,
58,
16,
25,
7857,
7,
47,
11,
16,
15437,
198,
437,
628,
198,
2,
22492,
5224,
694,
25855,
220,
198,
2,
383,
5224,
694,
4050,
26741,
389,
35560,
320,
1071,
453,
12,
8770,
1886,
4324,
5499,
18268,
416,
262,
220,
198,
2,
21543,
36237,
13,
770,
10361,
2163,
9743,
262,
5224,
694,
26741,
422,
262,
371,
3955,
46,
11,
543,
220,
198,
2,
389,
286,
262,
1296,
4600,
15751,
62,
17,
62,
15751,
47671,
4600,
15751,
62,
17,
62,
6500,
63,
3503,
13,
11680,
453,
11,
262,
5224,
694,
5444,
430,
389,
8574,
220,
198,
2,
351,
262,
40039,
286,
262,
15584,
12,
19816,
278,
17593,
5625,
13,
220,
198,
2,
198,
2,
7559,
63,
11018,
198,
2,
327,
23330,
59,
695,
92,
796,
370,
36796,
12,
16,
92,
23330,
59,
695,
92,
3467,
5183,
90,
34,
92,
23330,
59,
695,
92,
220,
198,
2,
7559,
63,
198,
198,
37811,
198,
220,
220,
220,
7736,
62,
11578,
694,
62,
40045,
62,
54,
75,
26933,
51,
3712,
6030,
28,
43879,
2414,
4357,
2030,
80,
16,
11,
6626,
16,
11,
2030,
80,
17,
11,
6626,
17,
11,
1020,
16,
62,
11,
1020,
17,
62,
26,
220,
198,
220,
220,
220,
220,
220,
220,
220,
300,
9806,
28,
27559,
11,
15584,
15908,
28,
22366,
8,
198,
198,
35561,
262,
5224,
694,
15584,
4351,
286,
685,
16684,
16,
60,
62,
1462,
62,
58,
16684,
17,
4357,
287,
370,
75,
1296,
13,
198,
198,
21017,
20559,
2886,
25,
198,
12,
4600,
51,
3712,
6030,
28,
43879,
2414,
63,
25,
11902,
717,
11507,
31577,
29052,
2099,
198,
12,
4600,
19503,
80,
16,
3712,
10100,
63,
25,
8373,
286,
717,
2214,
220,
198,
12,
4600,
35312,
16,
3712,
10100,
63,
25,
6626,
286,
717,
2214,
357,
72,
13,
68,
13,
289,
76,
16,
8,
198,
12,
4600,
19503,
80,
17,
3712,
10100,
63,
25,
8373,
286,
717,
2214,
220,
198,
12,
4600,
35312,
17,
3712,
10100,
63,
25,
6626,
286,
1218,
2214,
357,
72,
13,
68,
13,
289,
76,
17,
8,
198,
12,
4600,
16684,
16,
62,
63,
25,
11513,
284,
4731,
11,
2723,
10958,
588,
26653,
220,
198,
12,
4600,
16684,
17,
62,
63,
25,
11513,
284,
4731,
11,
10965,
10958,
198,
198,
21017,
7383,
10879,
198,
12,
4600,
75,
9806,
28,
27559,
63,
25,
5415,
18540,
2305,
198,
12,
4600,
40045,
15908,
28,
22366,
63,
25,
8619,
7268,
15584,
376,
29722,
3696,
13,
611,
2147,
11,
481,
2121,
736,
284,
220,
198,
220,
220,
220,
220,
220,
220,
220,
262,
4333,
49738,
430,
13,
20362,
15584,
3696,
13,
198,
198,
21017,
16409,
25,
220,
198,
12,
4600,
49738,
1373,
38469,
63,
25,
262,
15584,
370,
75,
11,
41497,
657,
25,
75,
9806,
198,
37811,
198,
8818,
7736,
62,
11578,
694,
62,
40045,
62,
54,
75,
7,
51,
3712,
6030,
11,
2030,
80,
16,
11,
6626,
16,
11,
2030,
80,
17,
11,
6626,
17,
11,
1020,
16,
62,
11,
1020,
17,
62,
26,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
300,
9806,
28,
27559,
11,
15584,
15908,
28,
22366,
8,
198,
220,
220,
220,
611,
318,
22366,
7,
40045,
15908,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
40539,
366,
40045,
8619,
407,
7368,
13,
15430,
284,
4333,
49738,
430,
13,
20362,
2121,
1891,
1,
198,
220,
220,
220,
220,
220,
220,
220,
15584,
15908,
796,
4333,
49738,
430,
13,
11578,
694,
11645,
62,
40045,
15908,
3419,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1020,
16,
796,
10903,
7,
16684,
16,
62,
8,
198,
220,
220,
220,
1020,
17,
796,
10903,
7,
16684,
17,
62,
8,
628,
220,
220,
220,
611,
21136,
7,
5317,
11,
2030,
80,
16,
8,
1875,
21136,
7,
5317,
11,
2030,
80,
17,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2030,
80,
16,
11,
2030,
80,
17,
796,
2030,
80,
17,
11,
2030,
80,
16,
198,
220,
220,
220,
220,
220,
220,
220,
6626,
16,
11,
6626,
17,
796,
6626,
17,
11,
6626,
16,
198,
220,
220,
220,
886,
198,
220,
220,
220,
611,
357,
19503,
80,
16,
6624,
2030,
80,
17,
8,
11405,
14808,
35312,
16,
6624,
366,
23940,
17,
4943,
11405,
357,
35312,
17,
6624,
366,
23940,
16,
48774,
198,
220,
220,
220,
220,
220,
220,
220,
6626,
16,
11,
6626,
17,
796,
6626,
17,
11,
6626,
16,
198,
220,
220,
220,
886,
628,
220,
220,
220,
277,
3672,
796,
366,
54,
75,
62,
49,
18,
13,
486,
62,
489,
1134,
27932,
62,
3,
7,
19503,
80,
16,
8,
3,
7,
35312,
16,
8,
87,
3,
7,
19503,
80,
17,
8,
3,
7,
35312,
17,
737,
21013,
1,
198,
220,
220,
220,
277,
796,
376,
29722,
7,
22179,
6978,
7,
40045,
15908,
11,
366,
3856,
321,
54,
69,
62,
39,
11674,
62,
49,
18,
13,
486,
1600,
277,
3672,
4008,
198,
220,
220,
220,
370,
75,
796,
10385,
7,
38469,
90,
51,
5512,
1100,
7,
69,
58,
16684,
16,
4357,
17971,
7,
16684,
16,
8,
62,
17,
62,
3,
7,
16684,
17,
8,
4943,
58,
45299,
16,
12962,
198,
220,
220,
220,
611,
300,
9806,
1279,
30123,
198,
220,
220,
220,
220,
220,
220,
220,
370,
75,
796,
370,
75,
58,
16,
25,
75,
9806,
10,
16,
60,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
370,
75,
796,
410,
9246,
7,
54,
75,
11,
938,
7,
54,
75,
8,
1635,
3392,
7,
51,
11,
300,
9806,
532,
30123,
4008,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
13058,
1373,
38469,
7,
54,
75,
8,
198,
437,
198,
22602,
62,
11578,
694,
62,
40045,
62,
54,
75,
7,
51,
3712,
6030,
11,
2030,
80,
16,
11,
6626,
16,
11,
2030,
80,
17,
11,
6626,
17,
11,
1020,
16,
26,
479,
86,
22046,
23029,
796,
220,
198,
220,
220,
220,
7736,
62,
11578,
694,
62,
40045,
62,
54,
75,
7,
51,
11,
2030,
80,
16,
11,
6626,
16,
11,
2030,
80,
17,
11,
6626,
17,
11,
1020,
16,
11,
1020,
16,
26,
479,
86,
22046,
23029,
198,
22602,
62,
11578,
694,
62,
40045,
62,
54,
75,
7,
19503,
80,
16,
3712,
10100,
11,
6626,
16,
11,
2030,
80,
17,
11,
6626,
17,
11,
1020,
16,
11,
1020,
17,
26,
479,
86,
22046,
23029,
796,
220,
198,
220,
220,
220,
7736,
62,
11578,
694,
62,
40045,
62,
54,
75,
7,
43879,
2414,
11,
2030,
80,
16,
11,
6626,
16,
11,
2030,
80,
17,
11,
6626,
17,
11,
1020,
16,
11,
1020,
17,
26,
479,
86,
22046,
23029,
628,
198,
2,
22492,
5224,
694,
4525,
11935,
17377,
82,
198,
2,
383,
5224,
694,
14955,
3544,
257,
2176,
3572,
286,
5444,
430,
290,
18540,
2305,
16069,
329,
883,
220,
198,
2,
5444,
430,
13,
775,
2148,
617,
10361,
5499,
284,
19818,
257,
4866,
286,
262,
5444,
430,
1502,
290,
220,
198,
2,
262,
18540,
2305,
5288,
290,
5415,
329,
883,
5444,
430,
13,
198,
198,
489,
291,
62,
2875,
3419,
796,
357,
198,
220,
220,
220,
357,
25,
15751,
553,
3064,
2430,
3064,
12340,
357,
25,
15751,
553,
21139,
2430,
21139,
12340,
357,
25,
15751,
553,
21139,
2430,
24591,
12340,
357,
25,
15751,
553,
24591,
2430,
24591,
12340,
220,
198,
220,
220,
220,
357,
25,
6500,
553,
3064,
2430,
3064,
12340,
357,
25,
6500,
553,
3064,
2430,
21139,
12340,
357,
25,
6500,
553,
3064,
2430,
24591,
12340,
357,
25,
6500,
553,
21139,
2430,
21139,
12340,
220,
198,
220,
220,
220,
357,
25,
6500,
553,
21139,
2430,
24591,
12340,
357,
25,
6500,
553,
24591,
2430,
24591,
12340,
220,
198,
220,
220,
220,
357,
25,
9328,
553,
3064,
2430,
3064,
12340,
357,
25,
9328,
553,
3064,
2430,
21139,
12340,
357,
25,
9328,
553,
3064,
2430,
24591,
12340,
357,
25,
9328,
553,
21139,
2430,
21139,
12340,
220,
198,
220,
220,
220,
357,
25,
9328,
553,
21139,
2430,
24591,
12340,
357,
25,
9328,
553,
24591,
2430,
24591,
4943,
198,
8,
198,
198,
9979,
458,
291,
62,
695,
81,
6231,
796,
360,
713,
7,
198,
220,
220,
220,
357,
25,
15751,
11,
366,
3064,
1600,
366,
3064,
4943,
5218,
357,
1270,
11,
15136,
22,
828,
198,
220,
220,
220,
357,
25,
15751,
11,
366,
21139,
1600,
366,
21139,
4943,
5218,
357,
1270,
11,
8235,
828,
198,
220,
220,
220,
357,
25,
15751,
11,
366,
21139,
1600,
366,
24591,
4943,
5218,
357,
1270,
11,
8646,
23,
828,
198,
220,
220,
220,
357,
25,
15751,
11,
366,
24591,
1600,
366,
24591,
4943,
5218,
357,
1270,
11,
8646,
23,
828,
198,
220,
220,
220,
357,
25,
6500,
11,
366,
3064,
1600,
366,
3064,
4943,
5218,
357,
1270,
11,
36006,
828,
198,
220,
220,
220,
357,
25,
6500,
11,
366,
3064,
1600,
366,
21139,
4943,
5218,
357,
1270,
11,
36006,
828,
198,
220,
220,
220,
357,
25,
6500,
11,
366,
3064,
1600,
366,
24591,
4943,
5218,
357,
31654,
11,
36006,
828,
198,
220,
220,
220,
357,
25,
6500,
11,
366,
21139,
1600,
366,
21139,
4943,
5218,
357,
1270,
11,
8235,
828,
198,
220,
220,
220,
357,
25,
6500,
11,
366,
21139,
1600,
366,
24591,
4943,
5218,
357,
31654,
11,
8235,
828,
198,
220,
220,
220,
357,
25,
6500,
11,
366,
24591,
1600,
366,
24591,
4943,
5218,
357,
31654,
11,
8235,
828,
628,
220,
220,
220,
357,
25,
9328,
11,
366,
3064,
1600,
366,
3064,
4943,
5218,
357,
1270,
11,
36006,
828,
198,
220,
220,
220,
357,
25,
9328,
11,
366,
3064,
1600,
366,
21139,
4943,
5218,
357,
1270,
11,
36006,
828,
198,
220,
220,
220,
357,
25,
9328,
11,
366,
3064,
1600,
366,
24591,
4943,
5218,
357,
31654,
11,
36006,
828,
198,
220,
220,
220,
357,
25,
9328,
11,
366,
21139,
1600,
366,
21139,
4943,
5218,
357,
1270,
11,
8235,
828,
198,
220,
220,
220,
357,
25,
9328,
11,
366,
21139,
1600,
366,
24591,
4943,
5218,
357,
31654,
11,
8235,
828,
198,
220,
220,
220,
357,
25,
9328,
11,
366,
24591,
1600,
366,
24591,
4943,
5218,
357,
31654,
11,
8235,
828,
198,
220,
220,
220,
220,
198,
220,
220,
220,
357,
25,
15751,
11,
366,
3064,
1600,
366,
21139,
4943,
5218,
357,
1270,
11,
36006,
828,
220,
220,
1303,
407,
973,
198,
220,
220,
220,
357,
25,
15751,
11,
366,
3064,
1600,
366,
24591,
4943,
5218,
357,
31654,
11,
36006,
828,
220,
1303,
407,
973,
198,
8,
198,
198,
8818,
651,
62,
489,
291,
62,
695,
9521,
7,
16684,
3712,
13940,
23650,
11,
2030,
80,
16,
11,
2030,
80,
17,
8,
198,
220,
220,
220,
611,
1020,
18872,
230,
357,
25,
9328,
11,
1058,
2767,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
21136,
7,
43879,
2414,
11,
2030,
80,
16,
8,
1875,
21136,
7,
43879,
2414,
11,
2030,
80,
17,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2030,
80,
16,
11,
2030,
80,
17,
796,
2030,
80,
17,
11,
2030,
80,
16,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
458,
291,
62,
695,
81,
6231,
58,
25,
9328,
11,
2030,
80,
16,
11,
2030,
80,
17,
60,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
458,
291,
62,
695,
81,
6231,
58,
16684,
11,
2030,
80,
16,
11,
2030,
80,
17,
60,
198,
437,
628,
198,
2,
22492,
26484,
13058,
430,
220,
198,
2,
383,
44829,
590,
17593,
17952,
290,
290,
6737,
27785,
2421,
281,
9672,
6737,
220,
198,
2,
5444,
430,
13,
775,
779,
262,
976,
36282,
5444,
430,
355,
973,
287,
262,
7559,
59,
5239,
90,
489,
291,
92,
15506,
14955,
13,
198,
2,
770,
5860,
48589,
3166,
329,
6737,
290,
4583,
7559,
34,
23330,
59,
695,
92,
15506,
287,
7559,
59,
30300,
59,
11018,
26224,
90,
42,
92,
15506,
220,
198,
2,
1022,
734,
19998,
13,
383,
1366,
318,
8574,
287,
262,
4600,
489,
291,
5420,
63,
8619,
287,
262,
4566,
13,
198,
198,
8818,
6737,
62,
392,
62,
1169,
652,
7,
19503,
80,
16,
11,
2030,
80,
17,
11,
4566,
3712,
35,
713,
8,
198,
220,
220,
220,
14955,
62,
7890,
62,
15908,
796,
4654,
6978,
7,
11250,
14692,
1416,
36722,
33116,
366,
489,
291,
5420,
4943,
198,
220,
220,
220,
294,
796,
1100,
62,
785,
12061,
62,
25677,
7,
22179,
6978,
7,
2339,
11935,
62,
7890,
62,
15908,
553,
1169,
652,
62,
565,
13,
14116,
48774,
198,
220,
220,
220,
277,
70,
796,
1100,
62,
785,
12061,
62,
25677,
7,
22179,
6978,
7,
2339,
11935,
62,
7890,
62,
15908,
11,
198,
220,
220,
220,
220,
220,
220,
220,
366,
8692,
62,
489,
1134,
36905,
62,
15751,
51,
31909,
62,
75,
4883,
62,
9319,
36,
62,
75,
26426,
13,
39504,
13,
489,
1134,
62,
754,
40520,
48774,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
329,
357,
16684,
11,
277,
16,
11,
277,
17,
8,
287,
458,
291,
62,
2875,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
300,
1084,
11,
300,
9806,
796,
651,
62,
489,
291,
62,
695,
9521,
7,
16684,
11,
277,
16,
11,
277,
17,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1500,
62,
2100,
796,
277,
70,
58,
75,
9806,
12,
16,
553,
3,
7,
16684,
8,
3,
7,
69,
16,
8,
55,
3,
7,
69,
17,
8,
8973,
198,
220,
220,
220,
220,
220,
220,
220,
22492,
6937,
36282,
1241,
706,
300,
9806,
1377,
612,
389,
15830,
20316,
4306,
198,
220,
220,
220,
220,
220,
220,
220,
277,
70,
58,
7,
75,
9806,
12,
16,
2599,
437,
11,
17971,
7,
16684,
8,
3,
7,
69,
16,
8,
55,
3,
7,
69,
17,
8,
8973,
764,
28,
1500,
62,
2100,
198,
220,
220,
220,
886,
628,
220,
220,
220,
22492,
9052,
625,
5444,
430,
290,
635,
6070,
287,
262,
26157,
1438,
198,
220,
220,
220,
2030,
48382,
796,
5855,
3064,
1600,
366,
21139,
1600,
366,
24591,
4943,
198,
220,
220,
220,
25274,
796,
5855,
15751,
1600,
366,
9328,
1600,
366,
2767,
1600,
366,
6500,
4943,
198,
220,
220,
220,
329,
277,
16,
287,
2030,
48382,
11,
277,
17,
287,
2030,
48382,
11,
1020,
287,
25274,
198,
220,
220,
220,
220,
220,
220,
220,
611,
17971,
7,
16684,
8,
3,
7,
69,
16,
8,
55,
3,
7,
69,
17,
16725,
18872,
231,
3891,
7,
40616,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
17971,
7,
50188,
7,
16684,
4008,
3,
7,
69,
17,
8,
55,
3,
7,
69,
16,
16725,
18872,
230,
3891,
7,
40616,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
277,
70,
58,
28265,
17971,
7,
16684,
8,
3,
7,
69,
16,
8,
55,
3,
7,
69,
17,
8,
8973,
796,
277,
70,
58,
28265,
17971,
7,
50188,
7,
16684,
4008,
3,
7,
69,
17,
8,
55,
3,
7,
69,
16,
8,
8973,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
277,
70,
58,
28265,
17971,
7,
16684,
8,
3,
7,
69,
16,
8,
55,
3,
7,
69,
17,
8,
8973,
796,
1976,
27498,
7,
77,
808,
7,
40616,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2471,
7,
85,
8,
796,
410,
9246,
26933,
15,
1539,
657,
13,
4357,
410,
8,
198,
220,
220,
220,
30004,
62,
38942,
796,
277,
70,
58,
28265,
366,
75,
8973,
764,
9,
357,
40616,
58,
28265,
366,
75,
8973,
764,
10,
352,
8,
24457,
357,
17,
46582,
1776,
198,
220,
220,
220,
6737,
62,
11600,
796,
360,
713,
90,
10100,
11,
38469,
90,
43879,
2414,
11709,
3419,
198,
220,
220,
220,
4583,
62,
11600,
796,
360,
713,
90,
10100,
11,
38469,
90,
43879,
2414,
11709,
3419,
628,
220,
220,
220,
329,
41420,
158,
224,
222,
287,
25274,
220,
22492,
41420,
158,
224,
222,
318,
262,
10958,
284,
3650,
198,
220,
220,
220,
220,
220,
220,
220,
277,
158,
224,
223,
11,
277,
158,
224,
224,
796,
21136,
7,
5317,
11,
2030,
80,
16,
828,
21136,
7,
5317,
11,
2030,
80,
17,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
277,
158,
224,
223,
19841,
277,
158,
224,
224,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
41420,
796,
41420,
158,
224,
222,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
220,
22492,
16075,
644,
356,
821,
2045,
329,
11,
355,
277,
70,
1366,
691,
468,
883,
3272,
12,
4443,
430,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
41420,
796,
41420,
158,
224,
222,
58,
17,
60,
1635,
41420,
158,
224,
222,
58,
16,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
277,
158,
224,
223,
11,
277,
158,
224,
224,
796,
277,
158,
224,
224,
11,
277,
158,
224,
223,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
611,
41420,
6624,
366,
2767,
1,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4583,
62,
565,
62,
34278,
796,
294,
58,
28265,
366,
9328,
8973,
24457,
357,
400,
58,
28265,
366,
43,
8973,
764,
9,
357,
400,
58,
28265,
366,
43,
8973,
764,
10,
352,
8,
24457,
357,
17,
46582,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4583,
62,
565,
62,
34278,
796,
294,
58,
28265,
41420,
60,
24457,
357,
400,
58,
28265,
366,
43,
8973,
764,
9,
357,
400,
58,
28265,
366,
43,
8973,
764,
10,
352,
8,
24457,
357,
17,
46582,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
277,
70,
62,
565,
62,
34278,
796,
277,
70,
58,
28265,
17971,
7,
34278,
8,
3,
7,
69,
158,
224,
223,
8,
55,
3,
7,
69,
158,
224,
224,
8,
8973,
24457,
357,
40616,
58,
28265,
366,
75,
8973,
764,
9,
357,
40616,
58,
28265,
366,
75,
8973,
764,
10,
352,
8,
24457,
357,
17,
46582,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
6737,
62,
11600,
58,
34278,
158,
224,
222,
60,
796,
220,
2471,
7,
1169,
652,
62,
565,
62,
34278,
764,
10,
277,
70,
62,
565,
62,
34278,
58,
16,
25,
9031,
22,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
4583,
62,
11600,
58,
34278,
158,
224,
222,
60,
796,
220,
2471,
7,
1169,
652,
62,
565,
62,
34278,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
6737,
62,
11600,
11,
4583,
62,
11600,
198,
437,
628,
198,
2,
22492,
5224,
694,
7559,
59,
5239,
926,
90,
489,
291,
92,
15506,
20984,
198,
2,
554,
2972,
3354,
286,
262,
11523,
11,
356,
765,
284,
8996,
674,
2482,
284,
262,
1743,
2864,
220,
198,
2,
1366,
2650,
13,
2312,
31878,
3440,
606,
422,
11898,
13,
1119,
821,
6338,
15680,
284,
262,
220,
198,
2,
4600,
489,
291,
5420,
63,
8619,
7368,
287,
262,
8398,
41526,
43,
13,
220,
628,
198,
37811,
198,
220,
220,
220,
5224,
694,
26687,
34,
709,
7,
489,
291,
5420,
6978,
3712,
10100,
8,
198,
198,
1273,
2850,
262,
5444,
430,
290,
39849,
3699,
728,
286,
262,
4941,
458,
291,
3781,
13,
198,
37811,
198,
20854,
694,
26687,
34,
709,
198,
198,
7249,
5224,
694,
26687,
34,
709,
90,
51,
92,
198,
220,
220,
220,
39849,
3712,
19182,
90,
51,
11,
17,
92,
198,
220,
220,
220,
30004,
82,
3712,
38469,
90,
5317,
92,
198,
220,
220,
220,
537,
82,
3712,
38469,
90,
51,
92,
198,
220,
220,
220,
8251,
3712,
38469,
90,
10100,
92,
198,
220,
220,
220,
850,
62,
521,
1063,
3712,
38469,
90,
5317,
92,
198,
220,
220,
220,
1994,
62,
9630,
62,
11600,
3712,
35,
713,
90,
10100,
11,
5317,
92,
198,
437,
198,
198,
8818,
5224,
694,
26687,
34,
709,
7,
489,
291,
5420,
6978,
8,
198,
220,
220,
220,
30004,
2777,
776,
796,
4654,
6978,
7,
489,
291,
5420,
6978,
11,
366,
35138,
62,
439,
62,
4443,
430,
13,
19608,
4943,
198,
220,
220,
220,
537,
6978,
796,
4654,
6978,
7,
489,
291,
5420,
6978,
11,
366,
7890,
62,
2302,
20216,
13,
19608,
4943,
198,
220,
220,
220,
39849,
6978,
796,
4654,
6978,
7,
489,
291,
5420,
6978,
11,
366,
66,
709,
6759,
13,
19608,
4943,
198,
220,
220,
220,
8251,
796,
14631,
15751,
62,
3064,
87,
3064,
1600,
366,
15751,
62,
21139,
87,
21139,
1600,
366,
15751,
62,
21139,
87,
24591,
1600,
366,
15751,
62,
24591,
87,
24591,
1600,
366,
6500,
62,
3064,
87,
3064,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
366,
6500,
62,
3064,
87,
21139,
1600,
366,
6500,
62,
3064,
87,
24591,
1600,
366,
6500,
62,
21139,
87,
21139,
1600,
366,
6500,
62,
21139,
87,
24591,
1600,
366,
6500,
62,
24591,
87,
24591,
1600,
366,
9328,
62,
3064,
87,
3064,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
366,
9328,
62,
3064,
87,
21139,
1600,
366,
9328,
62,
3064,
87,
24591,
1600,
366,
9328,
62,
21139,
87,
21139,
1600,
366,
9328,
62,
21139,
87,
24591,
1600,
366,
9328,
62,
24591,
87,
24591,
8973,
198,
220,
220,
220,
39849,
796,
800,
7,
961,
25404,
76,
7,
66,
709,
6978,
4008,
198,
220,
220,
220,
30004,
82,
796,
1100,
25404,
76,
7,
695,
2777,
776,
38381,
45299,
16,
60,
198,
220,
220,
220,
537,
82,
796,
1100,
25404,
76,
7,
565,
6978,
38381,
45299,
17,
60,
628,
220,
220,
220,
850,
18747,
62,
521,
1063,
796,
2824,
7,
15,
37498,
7857,
7,
66,
709,
11,
16,
13219,
17,
4008,
58,
19796,
439,
7,
26069,
7,
19187,
8,
764,
27,
657,
8,
764,
10,
352,
60,
764,
10,
352,
198,
220,
220,
220,
850,
62,
521,
1063,
796,
685,
16,
11,
850,
18747,
62,
521,
1063,
986,
11,
4129,
7,
565,
82,
47762,
16,
60,
198,
220,
220,
220,
1994,
62,
521,
796,
352,
25,
13664,
7,
13083,
8,
198,
220,
220,
220,
1994,
62,
9630,
62,
11600,
796,
360,
713,
7,
13083,
764,
14804,
1994,
62,
521,
8,
628,
220,
220,
220,
1441,
5224,
694,
26687,
34,
709,
90,
43879,
2414,
92,
7,
66,
709,
11,
30004,
82,
11,
537,
82,
11,
8251,
11,
850,
62,
521,
1063,
11,
1994,
62,
9630,
62,
11600,
8,
198,
437,
628,
198,
37811,
198,
220,
220,
220,
651,
62,
7266,
66,
709,
7,
489,
3712,
20854,
694,
26687,
34,
709,
11,
1020,
16,
11,
1020,
17,
8,
198,
198,
11627,
974,
262,
850,
12,
66,
709,
2743,
590,
17593,
11188,
284,
1020,
16,
13958,
1020,
17,
13,
198,
198,
21017,
20559,
2886,
25,
198,
12,
4600,
489,
3712,
20854,
694,
26687,
34,
709,
63,
25,
1366,
4645,
23069,
4941,
39849,
6759,
290,
5444,
430,
198,
12,
4600,
16684,
16,
3712,
10100,
63,
25,
10958,
286,
1296,
1312,
13,
68,
13,
366,
15751,
62,
3064,
87,
3064,
1,
198,
12,
4600,
16684,
17,
3712,
10100,
63,
25,
10958,
286,
1296,
1312,
13,
68,
13,
366,
15751,
62,
3064,
87,
3064,
1,
198,
198,
21017,
16409,
25,
220,
198,
12,
4600,
46912,
90,
43879,
2414,
92,
63,
25,
850,
66,
709,
2743,
590,
17593,
198,
37811,
198,
8818,
651,
62,
7266,
66,
709,
7,
489,
3712,
20854,
694,
26687,
34,
709,
11,
1020,
16,
11,
1020,
17,
8,
198,
220,
220,
220,
1312,
796,
458,
13,
2539,
62,
9630,
62,
11600,
58,
16684,
16,
60,
198,
220,
220,
220,
474,
796,
458,
13,
2539,
62,
9630,
62,
11600,
58,
16684,
17,
60,
198,
220,
220,
220,
1441,
458,
13,
66,
709,
58,
198,
220,
220,
220,
220,
220,
220,
220,
458,
13,
7266,
62,
521,
1063,
58,
72,
60,
1058,
357,
489,
13,
7266,
62,
521,
1063,
58,
72,
1343,
352,
60,
532,
352,
828,
198,
220,
220,
220,
220,
220,
220,
220,
458,
13,
7266,
62,
521,
1063,
58,
73,
60,
1058,
357,
489,
13,
7266,
62,
521,
1063,
58,
73,
1343,
352,
60,
532,
352,
828,
198,
220,
220,
220,
2361,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
7925,
62,
16684,
62,
392,
62,
66,
709,
7,
489,
3712,
20854,
694,
26687,
34,
709,
11,
1020,
16,
8,
198,
198,
11627,
974,
262,
4941,
30004,
82,
11,
537,
11,
4049,
5657,
11,
290,
850,
12,
66,
709,
2743,
590,
2512,
329,
257,
10958,
13958,
2346,
13,
198,
198,
21017,
20559,
2886,
25,
198,
12,
4600,
489,
3712,
20854,
694,
26687,
34,
709,
63,
25,
1366,
4645,
23069,
4941,
39849,
6759,
290,
5444,
430,
198,
12,
4600,
16684,
16,
3712,
10100,
63,
25,
10958,
286,
1296,
1312,
13,
68,
13,
366,
15751,
62,
3064,
87,
3064,
1,
198,
198,
21017,
16409,
25,
220,
198,
12,
4600,
7,
19187,
11,
537,
11,
11454,
11,
428,
62,
7266,
66,
709,
8,
63,
198,
37811,
198,
8818,
7925,
62,
16684,
62,
392,
62,
66,
709,
7,
489,
3712,
20854,
694,
26687,
34,
709,
11,
1020,
16,
8,
198,
220,
220,
220,
1312,
796,
458,
13,
2539,
62,
9630,
62,
11600,
58,
16684,
16,
60,
198,
220,
220,
220,
428,
62,
7266,
66,
709,
796,
651,
62,
7266,
66,
709,
7,
489,
11,
1020,
16,
11,
1020,
16,
8,
198,
220,
220,
220,
30004,
82,
796,
458,
13,
19187,
58,
489,
13,
7266,
62,
521,
1063,
58,
72,
5974,
7,
489,
13,
7266,
62,
521,
1063,
58,
72,
1343,
352,
60,
532,
352,
15437,
198,
220,
220,
220,
537,
796,
458,
13,
565,
82,
58,
489,
13,
7266,
62,
521,
1063,
58,
72,
5974,
7,
489,
13,
7266,
62,
521,
1063,
58,
72,
1343,
352,
60,
532,
352,
15437,
198,
220,
220,
220,
11454,
796,
19862,
17034,
12195,
10989,
363,
7,
5661,
62,
7266,
66,
709,
4008,
198,
220,
220,
220,
1441,
30004,
82,
11,
537,
11,
11454,
11,
428,
62,
7266,
66,
709,
198,
437,
198
] | 2.281256 | 4,871 |
using Revise
using AdFem
using PyCall
using LinearAlgebra
using PyPlot
using SparseArrays
using MAT
np = pyimport("numpy")
λ = 2.0
μ = 0.5
η = 1/12
β = 1/4; γ = 1/2
a = b = 0.1
m = 10
n = 5
h = 0.01
NT = 20
Δt = 1/NT
bdedge = []
for j = 1:n
push!(bdedge, [(j-1)*(m+1)+m+1 j*(m+1)+m+1])
end
bdedge = vcat(bdedge...)
bdnode = Int64[]
for j = 1:n+1
push!(bdnode, (j-1)*(m+1)+1)
end
G = [1/Δt+μ/η -μ/3η 0.0
-μ/3η 1/Δt+μ/η-μ/3η 0.0
0.0 0.0 1/Δt+μ/η]
S = [2μ/Δt+λ/Δt λ/Δt 0.0
λ/Δt 2μ/Δt+λ/Δt 0.0
0.0 0.0 μ/Δt]
invG = inv(G)
H = invG*S
M = compute_fem_mass_matrix1(m, n, h)
Zero = spzeros((m+1)*(n+1), (m+1)*(n+1))
M = [M Zero;Zero M]
K = compute_fem_stiffness_matrix(H, m, n, h)
C = a*M + b*K # damping matrix
L = M + γ*Δt*C + β*Δt^2*K
L, Lbd = fem_impose_Dirichlet_boundary_condition(L, bdnode, m, n, h)
Forces = zeros(NT, 2(m+1)*(n+1))
for i = 1:NT
T = eval_f_on_boundary_edge((x,y)->0.1, bdedge, m, n, h)
T = [T zeros(length(T))]
rhs = compute_fem_traction_term(T, bdedge, m, n, h)
if i*Δt>0.5
rhs = zero(rhs)
end
Forces[i, :] = rhs
end
a = zeros(2(m+1)*(n+1))
v = zeros(2(m+1)*(n+1))
d = zeros(2(m+1)*(n+1))
U = zeros(2(m+1)*(n+1),NT+1)
Sigma = zeros(NT+1, 4m*n, 3)
Varepsilon = zeros(NT+1, 4m*n, 3)
for i = 1:NT
@info i
global a, v, d
F = compute_strain_energy_term(Sigma[i,:,:]*invG/Δt, m, n, h) - K * U[:,i]
# @show norm(compute_strain_energy_term(Sigma[i,:,:]*invG/Δt, m, n, h)), norm(K * U[:,i])
rhs = Forces[i,:] - Δt^2 * F
td = d + Δt*v + Δt^2/2*(1-2β)*a
tv = v + (1-γ)*Δt*a
rhs = rhs - C*tv - K*td
rhs[[bdnode; bdnode.+(m+1)*(n+1)]] .= 0.0
a = L\rhs
d = td + β*Δt^2*a
v = tv + γ*Δt*a
U[:,i+1] = d
Varepsilon[i+1,:,:] = eval_strain_on_gauss_pts(U[:,i+1], m, n, h)
Sigma[i+1,:,:] = Sigma[i,:,:]*invG/Δt + (Varepsilon[i+1,:,:]-Varepsilon[i,:,:])*(invG*S)
end
matwrite("U.mat", Dict("U"=>U'|>Array))
# visualize_displacement(U, m, n, h; name = "_eta$η", xlim_=[-0.01,0.5], ylim_=[-0.05,0.22])
# visualize_displacement(U, m, n, h; name = "_viscoelasticity")
# visualize_stress(H, U, m, n, h; name = "_viscoelasticity")
close("all")
figure(figsize=(15,5))
subplot(1,3,1)
idx = div(n,2)*(m+1) + m+1
plot((0:NT)*Δt, U[idx,:])
xlabel("time")
ylabel("\$u_x\$")
subplot(1,3,2)
idx = 4*(div(n,2)*m + m)
plot((0:NT)*Δt, Sigma[:,idx,1])
xlabel("time")
ylabel("\$\\sigma_{xx}\$")
subplot(1,3,3)
idx = 4*(div(n,2)*m + m)
plot((0:NT)*Δt, Varepsilon[:,idx,1])
xlabel("time")
ylabel("\$\\varepsilon_{xx}\$")
savefig("visco_eta$η.png")
| [
3500,
5416,
786,
198,
3500,
1215,
37,
368,
198,
3500,
9485,
14134,
198,
3500,
44800,
2348,
29230,
198,
3500,
9485,
43328,
198,
3500,
1338,
17208,
3163,
20477,
198,
3500,
36775,
198,
37659,
796,
12972,
11748,
7203,
77,
32152,
4943,
198,
198,
39377,
796,
362,
13,
15,
198,
34703,
796,
657,
13,
20,
198,
138,
115,
796,
352,
14,
1065,
198,
198,
26638,
796,
352,
14,
19,
26,
7377,
111,
796,
352,
14,
17,
198,
64,
796,
275,
796,
657,
13,
16,
198,
76,
796,
838,
198,
77,
796,
642,
198,
71,
796,
657,
13,
486,
198,
11251,
796,
1160,
198,
138,
242,
83,
796,
352,
14,
11251,
220,
198,
65,
9395,
469,
796,
17635,
198,
1640,
474,
796,
352,
25,
77,
220,
198,
220,
4574,
0,
7,
65,
9395,
469,
11,
47527,
73,
12,
16,
27493,
7,
76,
10,
16,
47762,
76,
10,
16,
474,
9,
7,
76,
10,
16,
47762,
76,
10,
16,
12962,
198,
437,
198,
65,
9395,
469,
796,
410,
9246,
7,
65,
9395,
469,
23029,
198,
198,
17457,
17440,
796,
2558,
2414,
21737,
198,
1640,
474,
796,
352,
25,
77,
10,
16,
198,
220,
4574,
0,
7,
17457,
17440,
11,
357,
73,
12,
16,
27493,
7,
76,
10,
16,
47762,
16,
8,
198,
437,
198,
198,
38,
796,
685,
16,
14,
138,
242,
83,
10,
34703,
14,
138,
115,
532,
34703,
14,
18,
138,
115,
657,
13,
15,
198,
220,
532,
34703,
14,
18,
138,
115,
352,
14,
138,
242,
83,
10,
34703,
14,
138,
115,
12,
34703,
14,
18,
138,
115,
657,
13,
15,
198,
220,
657,
13,
15,
657,
13,
15,
352,
14,
138,
242,
83,
10,
34703,
14,
138,
115,
60,
198,
50,
796,
685,
17,
34703,
14,
138,
242,
83,
10,
39377,
14,
138,
242,
83,
7377,
119,
14,
138,
242,
83,
657,
13,
15,
198,
220,
220,
220,
7377,
119,
14,
138,
242,
83,
362,
34703,
14,
138,
242,
83,
10,
39377,
14,
138,
242,
83,
657,
13,
15,
198,
220,
220,
220,
657,
13,
15,
657,
13,
15,
18919,
14,
138,
242,
83,
60,
198,
16340,
38,
796,
800,
7,
38,
8,
198,
39,
796,
800,
38,
9,
50,
198,
198,
44,
796,
24061,
62,
69,
368,
62,
22208,
62,
6759,
8609,
16,
7,
76,
11,
299,
11,
289,
8,
198,
28667,
796,
599,
9107,
418,
19510,
76,
10,
16,
27493,
7,
77,
10,
16,
828,
357,
76,
10,
16,
27493,
7,
77,
10,
16,
4008,
198,
44,
796,
685,
44,
12169,
26,
28667,
337,
60,
198,
198,
42,
796,
24061,
62,
69,
368,
62,
301,
733,
1108,
62,
6759,
8609,
7,
39,
11,
285,
11,
299,
11,
289,
8,
198,
34,
796,
257,
9,
44,
1343,
275,
9,
42,
1303,
21151,
278,
17593,
220,
198,
198,
43,
796,
337,
1343,
7377,
111,
9,
138,
242,
83,
9,
34,
1343,
27169,
9,
138,
242,
83,
61,
17,
9,
42,
198,
43,
11,
406,
17457,
796,
2796,
62,
320,
3455,
62,
35277,
488,
1616,
62,
7784,
560,
62,
31448,
7,
43,
11,
275,
67,
17440,
11,
285,
11,
299,
11,
289,
8,
198,
198,
1890,
728,
796,
1976,
27498,
7,
11251,
11,
362,
7,
76,
10,
16,
27493,
7,
77,
10,
16,
4008,
198,
1640,
1312,
796,
352,
25,
11251,
198,
220,
309,
796,
5418,
62,
69,
62,
261,
62,
7784,
560,
62,
14907,
19510,
87,
11,
88,
8,
3784,
15,
13,
16,
11,
275,
9395,
469,
11,
285,
11,
299,
11,
289,
8,
198,
220,
309,
796,
685,
51,
1976,
27498,
7,
13664,
7,
51,
4008,
60,
198,
220,
9529,
82,
796,
24061,
62,
69,
368,
62,
83,
7861,
62,
4354,
7,
51,
11,
275,
9395,
469,
11,
285,
11,
299,
11,
289,
8,
628,
220,
611,
1312,
9,
138,
242,
83,
29,
15,
13,
20,
198,
220,
220,
220,
9529,
82,
796,
6632,
7,
81,
11994,
8,
198,
220,
886,
198,
220,
12700,
58,
72,
11,
1058,
60,
796,
9529,
82,
198,
437,
628,
198,
64,
796,
1976,
27498,
7,
17,
7,
76,
10,
16,
27493,
7,
77,
10,
16,
4008,
198,
85,
796,
1976,
27498,
7,
17,
7,
76,
10,
16,
27493,
7,
77,
10,
16,
4008,
198,
67,
796,
1976,
27498,
7,
17,
7,
76,
10,
16,
27493,
7,
77,
10,
16,
4008,
198,
52,
796,
1976,
27498,
7,
17,
7,
76,
10,
16,
27493,
7,
77,
10,
16,
828,
11251,
10,
16,
8,
198,
50,
13495,
796,
1976,
27498,
7,
11251,
10,
16,
11,
604,
76,
9,
77,
11,
513,
8,
198,
53,
533,
862,
33576,
796,
1976,
27498,
7,
11251,
10,
16,
11,
604,
76,
9,
77,
11,
513,
8,
198,
1640,
1312,
796,
352,
25,
11251,
220,
198,
220,
2488,
10951,
1312,
220,
198,
220,
220,
220,
3298,
257,
11,
410,
11,
288,
198,
220,
220,
220,
376,
796,
24061,
62,
2536,
391,
62,
22554,
62,
4354,
7,
50,
13495,
58,
72,
11,
45299,
47715,
9,
16340,
38,
14,
138,
242,
83,
11,
285,
11,
299,
11,
289,
8,
532,
509,
1635,
471,
58,
45299,
72,
60,
198,
220,
220,
220,
1303,
2488,
12860,
2593,
7,
5589,
1133,
62,
2536,
391,
62,
22554,
62,
4354,
7,
50,
13495,
58,
72,
11,
45299,
47715,
9,
16340,
38,
14,
138,
242,
83,
11,
285,
11,
299,
11,
289,
36911,
2593,
7,
42,
1635,
471,
58,
45299,
72,
12962,
198,
220,
220,
220,
9529,
82,
796,
12700,
58,
72,
11,
47715,
532,
37455,
83,
61,
17,
1635,
376,
628,
220,
220,
220,
41560,
796,
288,
1343,
37455,
83,
9,
85,
1343,
37455,
83,
61,
17,
14,
17,
9,
7,
16,
12,
17,
26638,
27493,
64,
220,
198,
220,
220,
220,
31557,
796,
410,
1343,
357,
16,
12,
42063,
27493,
138,
242,
83,
9,
64,
220,
198,
220,
220,
220,
9529,
82,
796,
9529,
82,
532,
327,
9,
14981,
532,
509,
9,
8671,
198,
220,
220,
220,
9529,
82,
30109,
17457,
17440,
26,
275,
67,
17440,
13,
33747,
76,
10,
16,
27493,
7,
77,
10,
16,
8,
11907,
764,
28,
657,
13,
15,
628,
220,
220,
220,
257,
796,
406,
59,
81,
11994,
220,
198,
220,
220,
220,
288,
796,
41560,
1343,
27169,
9,
138,
242,
83,
61,
17,
9,
64,
220,
198,
220,
220,
220,
410,
796,
31557,
1343,
7377,
111,
9,
138,
242,
83,
9,
64,
220,
198,
220,
220,
220,
471,
58,
45299,
72,
10,
16,
60,
796,
288,
628,
220,
220,
220,
569,
533,
862,
33576,
58,
72,
10,
16,
11,
45299,
47715,
796,
5418,
62,
2536,
391,
62,
261,
62,
4908,
1046,
62,
457,
82,
7,
52,
58,
45299,
72,
10,
16,
4357,
285,
11,
299,
11,
289,
8,
198,
220,
220,
220,
31669,
58,
72,
10,
16,
11,
45299,
47715,
796,
31669,
58,
72,
11,
45299,
47715,
9,
16340,
38,
14,
138,
242,
83,
1343,
220,
357,
53,
533,
862,
33576,
58,
72,
10,
16,
11,
45299,
25,
45297,
53,
533,
862,
33576,
58,
72,
11,
45299,
25,
12962,
9,
7,
16340,
38,
9,
50,
8,
198,
437,
198,
198,
6759,
13564,
7203,
52,
13,
6759,
1600,
360,
713,
7203,
52,
1,
14804,
52,
6,
91,
29,
19182,
4008,
628,
198,
2,
38350,
62,
6381,
489,
5592,
7,
52,
11,
285,
11,
299,
11,
289,
26,
1438,
796,
45434,
17167,
3,
138,
115,
1600,
2124,
2475,
62,
41888,
12,
15,
13,
486,
11,
15,
13,
20,
4357,
331,
2475,
62,
41888,
12,
15,
13,
2713,
11,
15,
13,
1828,
12962,
198,
2,
38350,
62,
6381,
489,
5592,
7,
52,
11,
285,
11,
299,
11,
289,
26,
220,
1438,
796,
45434,
4703,
1073,
417,
3477,
414,
4943,
198,
2,
38350,
62,
41494,
7,
39,
11,
471,
11,
285,
11,
299,
11,
289,
26,
220,
1438,
796,
45434,
4703,
1073,
417,
3477,
414,
4943,
198,
198,
19836,
7203,
439,
4943,
198,
26875,
7,
5647,
7857,
16193,
1314,
11,
20,
4008,
198,
7266,
29487,
7,
16,
11,
18,
11,
16,
8,
198,
312,
87,
796,
2659,
7,
77,
11,
17,
27493,
7,
76,
10,
16,
8,
1343,
285,
10,
16,
198,
29487,
19510,
15,
25,
11251,
27493,
138,
242,
83,
11,
471,
58,
312,
87,
11,
25,
12962,
198,
87,
18242,
7203,
2435,
4943,
198,
2645,
9608,
7203,
59,
3,
84,
62,
87,
59,
3,
4943,
198,
198,
7266,
29487,
7,
16,
11,
18,
11,
17,
8,
198,
312,
87,
796,
604,
9,
7,
7146,
7,
77,
11,
17,
27493,
76,
1343,
285,
8,
198,
29487,
19510,
15,
25,
11251,
27493,
138,
242,
83,
11,
31669,
58,
45299,
312,
87,
11,
16,
12962,
198,
87,
18242,
7203,
2435,
4943,
198,
2645,
9608,
7203,
59,
3,
6852,
82,
13495,
23330,
5324,
32239,
3,
4943,
198,
198,
7266,
29487,
7,
16,
11,
18,
11,
18,
8,
198,
312,
87,
796,
604,
9,
7,
7146,
7,
77,
11,
17,
27493,
76,
1343,
285,
8,
198,
29487,
19510,
15,
25,
11251,
27493,
138,
242,
83,
11,
569,
533,
862,
33576,
58,
45299,
312,
87,
11,
16,
12962,
198,
87,
18242,
7203,
2435,
4943,
198,
2645,
9608,
7203,
59,
3,
6852,
85,
533,
862,
33576,
23330,
5324,
32239,
3,
4943,
198,
21928,
5647,
7203,
4703,
1073,
62,
17167,
3,
138,
115,
13,
11134,
4943,
198
] | 1.679868 | 1,515 |
"""
Cell(;has_mine=false, flagged=false, revealed=false, mine_count=0, idx=(0,0))
Cell represents the state of a cell in Minesweeper
- `has_mine`: true if cell contains a mine
- `flagged`: true if cell has been flagged as having a mine
- `revealed`: true if the cell has been revealed
- `mine_count`: number of mines in adjecent cells
- `idx`: Cartesian indices of cell
"""
mutable struct Cell
has_mine::Bool
flagged::Bool
revealed::Bool
mine_count::Int
idx::CartesianIndex
end
function Cell(;has_mine=false, flagged=false, revealed=false, mine_count=0, idx=(0,0))
coords = CartesianIndex(idx)
return Cell(has_mine, flagged, revealed, mine_count, coords)
end
"""
Game(;dims=(12,12), n_mines=40, mines_flagged=0, mine_detonated=false, trials=0)
Minesweeper game object
- `cells`: an array of cells
- `dims`: a Tuple indicating dimensions of cell array
- `n_mines`: number of mines in the game
- `mines_flagged`: number of mines flagged
- `mine_detonated`: indicates whether a mine has been detonated
- `score`: score for game, which includes hits, misses, false alarms and correct rejections
- `trials`: the number of trials or moves
"""
mutable struct Game{T}
cells::Array{Cell,2}
dims::Tuple{Int,Int}
n_mines::Int
mines_flagged::Int
mine_detonated::Bool
score::T
trials::Int
end
function Game(;dims=(12,12), n_mines=40, mines_flagged=0,
mine_detonated=false, trials=0)
score = (hits=0.0,false_alarms=0.0,misses=0.0,correct_rejections=0.0)
cells = initilize_cells(dims)
add_mines!(cells, n_mines)
mine_count!(cells)
return Game(cells, dims, n_mines, mines_flagged, mine_detonated,
score, trials)
end
function initilize_cells(dims)
return [Cell(idx=(r,c)) for r in 1:dims[1], c in 1:dims[2]]
end
function add_mines!(cells, n_mines)
mines = sample(cells, n_mines, replace=false)
for m in mines
m.has_mine = true
end
return nothing
end
"""
mine_count!(cells)
For each cell, omputes the number of neighboring cells containing a mine.
"""
function mine_count!(cells)
for cell in cells
neighbors = get_neighbors(cells, cell.idx)
cell.mine_count = count(x->x.has_mine, neighbors)
end
return nothing
end
get_neighbors(game::Game, idx) = get_neighbors(game.cells, idx.I...)
get_neighbors(game::Game, cell::Cell) = get_neighbors(game.cells, cell.idx.I...)
get_neighbors(game::Game, x, y) = get_neighbors(game.cells, x, y)
get_neighbors(cells, idx) = get_neighbors(cells, idx.I...)
function get_neighbors(cells, xr, yr)
v = [-1,0,1]
X = xr .+ v; Y = yr .+ v
neighbors = Vector{Cell}()
for y in Y, x in X
(x == xr) && (y == yr) ? (continue) : nothing
in_bounds(cells, x, y) ? push!(neighbors, cells[x,y]) : nothing
end
return neighbors
end
get_neighbor_indices(game::Game, idx) = get_neighbor_indices(game.cells, idx.I...)
get_neighbor_indices(game::Game, x, y) = get_neighbor_indices(game.cells, x, y)
get_neighbor_indices(cells, idx) = get_neighbor_indices(cells, idx.I...)
function get_neighbor_indices(cells, xr, yr)
v = [-1,0,1]
X = xr .+ v; Y = yr .+ v
neighbors = Vector{CartesianIndex}()
for y in Y, x in X
(x == xr) && (y == yr) ? (continue) : nothing
in_bounds(cells, x, y) ? push!(neighbors, CartesianIndex(x,y)) : nothing
end
return neighbors
end
function in_bounds(cells, x, y)
if (x < 1) || (y < 1)
return false
elseif (x > size(cells, 1)) || (y > size(cells, 2))
return false
else
return true
end
end
reveal_zeros!(game::Game, x, y) = reveal_zeros!(game.cells, CartesianIndex(x, y))
reveal_zeros!(game::Game, idx) = reveal_zeros!(game.cells, idx)
function reveal_zeros!(cells, idx)
cells[idx].mine_count ≠ 0 ? (return nothing) : nothing
indices = get_neighbor_indices(cells, idx)
for i in indices
c = cells[i]
if !c.has_mine && !c.revealed
c.revealed = true
c.mine_count == 0 ? reveal_zeros!(cells, i) : nothing
end
end
return nothing
end
reveal(game::Game) = reveal(game.cells::Array{Cell,2})
"""
reveal(cells::Array{Cell,2})
Reveals game state in REPL
"""
function reveal(cells::Array{Cell,2})
s = ""
for x in 1:size(cells,1)
for y in 1:size(cells,2)
c = cells[x,y]
if c.has_mine
s *= string(" ", "💣", " ")
else
s *= string(" ", c.mine_count, " ")
end
end
s *= "\r\n"
end
println(s)
end
Base.show(io::IO, game::Game) = Base.show(io::IO, game.cells)
function Base.show(io::IO, cells::Array{Cell,2})
s = ""
for x in 1:size(cells,1)
for y in 1:size(cells,2)
c = cells[x,y]
if c.flagged
s *= string(" ", "🚩", " ")
continue
end
if c.revealed
if c.has_mine
s *= string(" ", "💣", " ")
continue
end
if c.mine_count == 0
s *= string(" ", "∘", " ")
else
s *= string(" ", c.mine_count, " ")
end
else
s *= string(" ", "■", " ")
end
end
s *= "\r\n"
end
println(s)
end
"""
game_over(game)
Terminates game if mine is detonated or all cells have been revealed or flagged.
"""
function game_over(game)
if game.mine_detonated
return true
end
return all(x->x.revealed || x.flagged, game.cells)
end
"""
compute_score!(game)
Computes hits, false alarms, misses and correct rejections
"""
function compute_score!(game)
cells = game.cells
n_mines = game.n_mines
no_mines = prod(game.dims) - n_mines
hits = count(x->x.flagged && x.has_mine, cells)
misses = n_mines - hits
false_alarms = count(x->x.flagged && !x.has_mine, cells)
cr = no_mines - false_alarms
game.score = (hits=hits,false_alarms=false_alarms,misses=misses,correct_rejections=cr)
return nothing
end
"""
select_cell!(game, x, y)
Select cell given a game and row and column indices.
"""
select_cell!(game, x, y) = select_cell!(game, CartesianIndex(x, y))
"""
select_cell!(game, x, y)
Select cell given a game and cell object.
"""
select_cell!(game, cell::Cell) = select_cell!(game, cell.idx)
"""
select_cell!(game, idx)
Select cell given a game and Cartesian index.
"""
function select_cell!(game, idx)
cell = game.cells[idx]
cell.revealed = true
reveal_zeros!(game, idx)
game.mine_detonated = cell.has_mine
return nothing
end
function flag_cell!(cell, game, gui::Nothing)
cell.flagged = true
game.mines_flagged += 1
return nothing
end
function setup()
println("Select Difficulty")
waiting = true
levels = [20,50,70]
i = 0
while waiting
d = input("1: easy 2: medium, 3: difficult")
i = parse(Int, d)
if i ∉ 1:3
println("Please select 1, 2, or 3")
else
waiting = false
end
end
return Game(;dims=(15,15), n_mines=levels[i])
end
function play()
game = setup()
play(game)
end
"""
play(game)
Play Minesweeper in REPL
Run play() to use with default easy, medium and difficult settings
Pass game object to play custom game
Enter exit to quit
Enter row_index column_index to select cell (e.g. 1 2)
"""
function play(game)
playing = true
coords = fill(0, 2)
println(game)
while playing
str = input("select coordinates")
str == "exit" ? (return) : nothing
try
coords = parse.(Int, split(str, " "))
catch
error("Please give x and y coordinates e.g. 3 2")
end
select_cell!(game, coords...)
if game.mine_detonated
reveal(game)
println("Game Over")
println(" ")
d = input("Press y to play again")
d == "y" ? (game = setup()) : (playing = false)
else
println(game)
end
end
end
function input(prompt)
println(prompt)
return readline()
end
"""
update!(game, gui::Nothing)
`update!` is used to display game state during simulations.
`update!` can be used with REPL or Gtk GUI if imported via import_gui()
"""
function update!(game, gui::Nothing)
println(game)
end
"""
imports Gtk related code for the GUI
"""
function import_gui()
path = @__DIR__
include(path*"/Gtk_Gui.jl")
end
| [
37811,
198,
220,
220,
220,
12440,
7,
26,
10134,
62,
3810,
28,
9562,
11,
34060,
28,
9562,
11,
4602,
28,
9562,
11,
6164,
62,
9127,
28,
15,
11,
4686,
87,
16193,
15,
11,
15,
4008,
198,
198,
28780,
6870,
262,
1181,
286,
257,
2685,
287,
33466,
732,
5723,
198,
12,
4600,
10134,
62,
3810,
63,
25,
2081,
611,
2685,
4909,
257,
6164,
198,
12,
4600,
2704,
14655,
63,
25,
2081,
611,
2685,
468,
587,
34060,
355,
1719,
257,
6164,
198,
12,
4600,
36955,
3021,
63,
25,
2081,
611,
262,
2685,
468,
587,
4602,
198,
12,
4600,
3810,
62,
9127,
63,
25,
1271,
286,
18446,
287,
9224,
721,
298,
4778,
198,
12,
4600,
312,
87,
63,
25,
13690,
35610,
36525,
286,
2685,
198,
37811,
198,
76,
18187,
2878,
12440,
198,
220,
220,
220,
468,
62,
3810,
3712,
33,
970,
198,
220,
220,
220,
34060,
3712,
33,
970,
198,
220,
220,
220,
4602,
3712,
33,
970,
198,
220,
220,
220,
6164,
62,
9127,
3712,
5317,
198,
220,
220,
220,
4686,
87,
3712,
43476,
35610,
15732,
198,
437,
198,
198,
8818,
12440,
7,
26,
10134,
62,
3810,
28,
9562,
11,
34060,
28,
9562,
11,
4602,
28,
9562,
11,
6164,
62,
9127,
28,
15,
11,
4686,
87,
16193,
15,
11,
15,
4008,
198,
220,
220,
220,
763,
3669,
796,
13690,
35610,
15732,
7,
312,
87,
8,
198,
220,
220,
220,
1441,
12440,
7,
10134,
62,
3810,
11,
34060,
11,
4602,
11,
6164,
62,
9127,
11,
763,
3669,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
3776,
7,
26,
67,
12078,
16193,
1065,
11,
1065,
828,
299,
62,
1084,
274,
28,
1821,
11,
18446,
62,
2704,
14655,
28,
15,
11,
6164,
62,
15255,
261,
515,
28,
9562,
11,
9867,
28,
15,
8,
198,
198,
44,
1127,
732,
5723,
983,
2134,
198,
198,
12,
4600,
46342,
63,
25,
281,
7177,
286,
4778,
198,
12,
4600,
67,
12078,
63,
25,
257,
309,
29291,
12739,
15225,
286,
2685,
7177,
198,
12,
4600,
77,
62,
1084,
274,
63,
25,
1271,
286,
18446,
287,
262,
983,
198,
12,
4600,
1084,
274,
62,
2704,
14655,
63,
25,
1271,
286,
18446,
34060,
198,
12,
4600,
3810,
62,
15255,
261,
515,
63,
25,
9217,
1771,
257,
6164,
468,
587,
38754,
198,
12,
4600,
26675,
63,
25,
4776,
329,
983,
11,
543,
3407,
7127,
11,
18297,
11,
3991,
36302,
290,
3376,
4968,
507,
198,
12,
4600,
28461,
874,
63,
25,
262,
1271,
286,
9867,
393,
6100,
198,
37811,
198,
76,
18187,
2878,
3776,
90,
51,
92,
198,
220,
220,
220,
4778,
3712,
19182,
90,
28780,
11,
17,
92,
198,
220,
220,
220,
5391,
82,
3712,
51,
29291,
90,
5317,
11,
5317,
92,
198,
220,
220,
220,
299,
62,
1084,
274,
3712,
5317,
198,
220,
220,
220,
18446,
62,
2704,
14655,
3712,
5317,
198,
220,
220,
220,
6164,
62,
15255,
261,
515,
3712,
33,
970,
198,
220,
220,
220,
4776,
3712,
51,
198,
220,
220,
220,
9867,
3712,
5317,
198,
437,
198,
198,
8818,
3776,
7,
26,
67,
12078,
16193,
1065,
11,
1065,
828,
299,
62,
1084,
274,
28,
1821,
11,
18446,
62,
2704,
14655,
28,
15,
11,
198,
220,
220,
220,
6164,
62,
15255,
261,
515,
28,
9562,
11,
9867,
28,
15,
8,
198,
220,
220,
220,
4776,
796,
357,
71,
896,
28,
15,
13,
15,
11,
9562,
62,
282,
8357,
28,
15,
13,
15,
11,
3927,
274,
28,
15,
13,
15,
11,
30283,
62,
260,
752,
507,
28,
15,
13,
15,
8,
198,
220,
220,
220,
4778,
796,
2315,
346,
1096,
62,
46342,
7,
67,
12078,
8,
198,
220,
220,
220,
751,
62,
1084,
274,
0,
7,
46342,
11,
299,
62,
1084,
274,
8,
198,
220,
220,
220,
6164,
62,
9127,
0,
7,
46342,
8,
198,
220,
220,
220,
1441,
3776,
7,
46342,
11,
5391,
82,
11,
299,
62,
1084,
274,
11,
18446,
62,
2704,
14655,
11,
6164,
62,
15255,
261,
515,
11,
198,
220,
220,
220,
220,
220,
220,
220,
4776,
11,
9867,
8,
198,
437,
198,
198,
8818,
2315,
346,
1096,
62,
46342,
7,
67,
12078,
8,
198,
220,
220,
220,
1441,
685,
28780,
7,
312,
87,
16193,
81,
11,
66,
4008,
329,
374,
287,
352,
25,
67,
12078,
58,
16,
4357,
269,
287,
352,
25,
67,
12078,
58,
17,
11907,
198,
437,
198,
198,
8818,
751,
62,
1084,
274,
0,
7,
46342,
11,
299,
62,
1084,
274,
8,
198,
220,
220,
220,
18446,
796,
6291,
7,
46342,
11,
299,
62,
1084,
274,
11,
6330,
28,
9562,
8,
198,
220,
220,
220,
329,
285,
287,
18446,
198,
220,
220,
220,
220,
220,
220,
220,
285,
13,
10134,
62,
3810,
796,
2081,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
2147,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
6164,
62,
9127,
0,
7,
46342,
8,
198,
198,
1890,
1123,
2685,
11,
267,
3149,
1769,
262,
1271,
286,
19651,
4778,
7268,
257,
6164,
13,
198,
37811,
198,
8818,
6164,
62,
9127,
0,
7,
46342,
8,
198,
220,
220,
220,
329,
2685,
287,
4778,
198,
220,
220,
220,
220,
220,
220,
220,
12020,
796,
651,
62,
710,
394,
32289,
7,
46342,
11,
2685,
13,
312,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2685,
13,
3810,
62,
9127,
796,
954,
7,
87,
3784,
87,
13,
10134,
62,
3810,
11,
12020,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
2147,
198,
437,
198,
198,
1136,
62,
710,
394,
32289,
7,
6057,
3712,
8777,
11,
4686,
87,
8,
796,
651,
62,
710,
394,
32289,
7,
6057,
13,
46342,
11,
4686,
87,
13,
40,
23029,
198,
1136,
62,
710,
394,
32289,
7,
6057,
3712,
8777,
11,
2685,
3712,
28780,
8,
796,
651,
62,
710,
394,
32289,
7,
6057,
13,
46342,
11,
2685,
13,
312,
87,
13,
40,
23029,
198,
1136,
62,
710,
394,
32289,
7,
6057,
3712,
8777,
11,
2124,
11,
331,
8,
796,
651,
62,
710,
394,
32289,
7,
6057,
13,
46342,
11,
2124,
11,
331,
8,
198,
1136,
62,
710,
394,
32289,
7,
46342,
11,
4686,
87,
8,
796,
651,
62,
710,
394,
32289,
7,
46342,
11,
4686,
87,
13,
40,
23029,
198,
198,
8818,
651,
62,
710,
394,
32289,
7,
46342,
11,
2124,
81,
11,
42635,
8,
198,
220,
220,
220,
410,
796,
25915,
16,
11,
15,
11,
16,
60,
198,
220,
220,
220,
1395,
796,
2124,
81,
764,
10,
410,
26,
575,
796,
42635,
764,
10,
410,
198,
220,
220,
220,
12020,
796,
20650,
90,
28780,
92,
3419,
198,
220,
220,
220,
329,
331,
287,
575,
11,
2124,
287,
1395,
198,
220,
220,
220,
220,
220,
220,
220,
357,
87,
6624,
2124,
81,
8,
11405,
357,
88,
6624,
42635,
8,
5633,
357,
43043,
8,
1058,
2147,
198,
220,
220,
220,
220,
220,
220,
220,
287,
62,
65,
3733,
7,
46342,
11,
2124,
11,
331,
8,
5633,
4574,
0,
7,
710,
394,
32289,
11,
4778,
58,
87,
11,
88,
12962,
1058,
2147,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
12020,
198,
437,
198,
198,
1136,
62,
710,
394,
2865,
62,
521,
1063,
7,
6057,
3712,
8777,
11,
4686,
87,
8,
796,
651,
62,
710,
394,
2865,
62,
521,
1063,
7,
6057,
13,
46342,
11,
4686,
87,
13,
40,
23029,
198,
1136,
62,
710,
394,
2865,
62,
521,
1063,
7,
6057,
3712,
8777,
11,
2124,
11,
331,
8,
796,
651,
62,
710,
394,
2865,
62,
521,
1063,
7,
6057,
13,
46342,
11,
2124,
11,
331,
8,
198,
1136,
62,
710,
394,
2865,
62,
521,
1063,
7,
46342,
11,
4686,
87,
8,
796,
651,
62,
710,
394,
2865,
62,
521,
1063,
7,
46342,
11,
4686,
87,
13,
40,
23029,
198,
198,
8818,
651,
62,
710,
394,
2865,
62,
521,
1063,
7,
46342,
11,
2124,
81,
11,
42635,
8,
198,
220,
220,
220,
410,
796,
25915,
16,
11,
15,
11,
16,
60,
198,
220,
220,
220,
1395,
796,
2124,
81,
764,
10,
410,
26,
575,
796,
42635,
764,
10,
410,
198,
220,
220,
220,
12020,
796,
20650,
90,
43476,
35610,
15732,
92,
3419,
198,
220,
220,
220,
329,
331,
287,
575,
11,
2124,
287,
1395,
198,
220,
220,
220,
220,
220,
220,
220,
357,
87,
6624,
2124,
81,
8,
11405,
357,
88,
6624,
42635,
8,
5633,
357,
43043,
8,
1058,
2147,
198,
220,
220,
220,
220,
220,
220,
220,
287,
62,
65,
3733,
7,
46342,
11,
2124,
11,
331,
8,
5633,
4574,
0,
7,
710,
394,
32289,
11,
13690,
35610,
15732,
7,
87,
11,
88,
4008,
1058,
2147,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
12020,
198,
437,
198,
198,
8818,
287,
62,
65,
3733,
7,
46342,
11,
2124,
11,
331,
8,
198,
220,
220,
220,
611,
357,
87,
1279,
352,
8,
8614,
357,
88,
1279,
352,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
3991,
198,
220,
220,
220,
2073,
361,
357,
87,
1875,
2546,
7,
46342,
11,
352,
4008,
8614,
357,
88,
1875,
2546,
7,
46342,
11,
362,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
3991,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
2081,
198,
220,
220,
220,
886,
198,
437,
198,
198,
36955,
282,
62,
9107,
418,
0,
7,
6057,
3712,
8777,
11,
2124,
11,
331,
8,
796,
7766,
62,
9107,
418,
0,
7,
6057,
13,
46342,
11,
13690,
35610,
15732,
7,
87,
11,
331,
4008,
198,
36955,
282,
62,
9107,
418,
0,
7,
6057,
3712,
8777,
11,
4686,
87,
8,
796,
7766,
62,
9107,
418,
0,
7,
6057,
13,
46342,
11,
4686,
87,
8,
198,
198,
8818,
7766,
62,
9107,
418,
0,
7,
46342,
11,
4686,
87,
8,
198,
220,
220,
220,
4778,
58,
312,
87,
4083,
3810,
62,
9127,
15139,
254,
657,
5633,
357,
7783,
2147,
8,
1058,
2147,
198,
220,
220,
220,
36525,
796,
651,
62,
710,
394,
2865,
62,
521,
1063,
7,
46342,
11,
4686,
87,
8,
198,
220,
220,
220,
329,
1312,
287,
36525,
198,
220,
220,
220,
220,
220,
220,
220,
269,
796,
4778,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
611,
5145,
66,
13,
10134,
62,
3810,
11405,
5145,
66,
13,
36955,
3021,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
13,
36955,
3021,
796,
2081,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
13,
3810,
62,
9127,
6624,
657,
5633,
7766,
62,
9107,
418,
0,
7,
46342,
11,
1312,
8,
1058,
2147,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
2147,
198,
437,
198,
198,
36955,
282,
7,
6057,
3712,
8777,
8,
796,
7766,
7,
6057,
13,
46342,
3712,
19182,
90,
28780,
11,
17,
30072,
198,
198,
37811,
198,
220,
220,
220,
7766,
7,
46342,
3712,
19182,
90,
28780,
11,
17,
30072,
198,
198,
3041,
303,
874,
983,
1181,
287,
45285,
198,
37811,
198,
8818,
7766,
7,
46342,
3712,
19182,
90,
28780,
11,
17,
30072,
198,
220,
220,
220,
264,
796,
13538,
198,
220,
220,
220,
329,
2124,
287,
352,
25,
7857,
7,
46342,
11,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
329,
331,
287,
352,
25,
7857,
7,
46342,
11,
17,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
796,
4778,
58,
87,
11,
88,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
269,
13,
10134,
62,
3810,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
264,
1635,
28,
4731,
7203,
33172,
366,
8582,
240,
96,
1600,
366,
366,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
264,
1635,
28,
4731,
7203,
33172,
269,
13,
3810,
62,
9127,
11,
366,
366,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
264,
1635,
28,
37082,
81,
59,
77,
1,
198,
220,
220,
220,
886,
198,
220,
220,
220,
44872,
7,
82,
8,
198,
437,
198,
198,
14881,
13,
12860,
7,
952,
3712,
9399,
11,
983,
3712,
8777,
8,
796,
7308,
13,
12860,
7,
952,
3712,
9399,
11,
983,
13,
46342,
8,
198,
198,
8818,
7308,
13,
12860,
7,
952,
3712,
9399,
11,
4778,
3712,
19182,
90,
28780,
11,
17,
30072,
198,
220,
220,
220,
264,
796,
13538,
198,
220,
220,
220,
329,
2124,
287,
352,
25,
7857,
7,
46342,
11,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
329,
331,
287,
352,
25,
7857,
7,
46342,
11,
17,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
796,
4778,
58,
87,
11,
88,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
269,
13,
2704,
14655,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
264,
1635,
28,
4731,
7203,
33172,
366,
8582,
248,
102,
1600,
366,
366,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2555,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
269,
13,
36955,
3021,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
269,
13,
10134,
62,
3810,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
264,
1635,
28,
4731,
7203,
33172,
366,
8582,
240,
96,
1600,
366,
366,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2555,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
269,
13,
3810,
62,
9127,
6624,
657,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
264,
1635,
28,
4731,
7203,
33172,
366,
24861,
246,
1600,
366,
366,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
264,
1635,
28,
4731,
7203,
33172,
269,
13,
3810,
62,
9127,
11,
366,
366,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
264,
1635,
28,
4731,
7203,
33172,
366,
29316,
1600,
366,
366,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
264,
1635,
28,
37082,
81,
59,
77,
1,
198,
220,
220,
220,
886,
198,
220,
220,
220,
44872,
7,
82,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
983,
62,
2502,
7,
6057,
8,
198,
198,
44798,
689,
983,
611,
6164,
318,
38754,
393,
477,
4778,
423,
587,
4602,
393,
34060,
13,
198,
37811,
198,
8818,
983,
62,
2502,
7,
6057,
8,
198,
220,
220,
220,
611,
983,
13,
3810,
62,
15255,
261,
515,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
2081,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
477,
7,
87,
3784,
87,
13,
36955,
3021,
8614,
2124,
13,
2704,
14655,
11,
983,
13,
46342,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
24061,
62,
26675,
0,
7,
6057,
8,
198,
198,
7293,
1769,
7127,
11,
3991,
36302,
11,
18297,
290,
3376,
4968,
507,
198,
37811,
198,
8818,
24061,
62,
26675,
0,
7,
6057,
8,
198,
220,
220,
220,
4778,
796,
983,
13,
46342,
198,
220,
220,
220,
299,
62,
1084,
274,
796,
983,
13,
77,
62,
1084,
274,
198,
220,
220,
220,
645,
62,
1084,
274,
796,
40426,
7,
6057,
13,
67,
12078,
8,
532,
299,
62,
1084,
274,
198,
220,
220,
220,
7127,
796,
954,
7,
87,
3784,
87,
13,
2704,
14655,
11405,
2124,
13,
10134,
62,
3810,
11,
4778,
8,
198,
220,
220,
220,
18297,
796,
299,
62,
1084,
274,
532,
7127,
198,
220,
220,
220,
3991,
62,
282,
8357,
796,
954,
7,
87,
3784,
87,
13,
2704,
14655,
11405,
5145,
87,
13,
10134,
62,
3810,
11,
4778,
8,
198,
220,
220,
220,
1067,
796,
645,
62,
1084,
274,
532,
3991,
62,
282,
8357,
198,
220,
220,
220,
983,
13,
26675,
796,
357,
71,
896,
28,
71,
896,
11,
9562,
62,
282,
8357,
28,
9562,
62,
282,
8357,
11,
3927,
274,
28,
3927,
274,
11,
30283,
62,
260,
752,
507,
28,
6098,
8,
198,
220,
220,
220,
1441,
2147,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
2922,
62,
3846,
0,
7,
6057,
11,
2124,
11,
331,
8,
198,
220,
220,
220,
220,
198,
17563,
2685,
1813,
257,
983,
290,
5752,
290,
5721,
36525,
13,
198,
37811,
198,
19738,
62,
3846,
0,
7,
6057,
11,
2124,
11,
331,
8,
796,
2922,
62,
3846,
0,
7,
6057,
11,
13690,
35610,
15732,
7,
87,
11,
331,
4008,
198,
198,
37811,
198,
220,
220,
220,
2922,
62,
3846,
0,
7,
6057,
11,
2124,
11,
331,
8,
198,
220,
220,
220,
220,
198,
17563,
2685,
1813,
257,
983,
290,
2685,
2134,
13,
198,
37811,
198,
19738,
62,
3846,
0,
7,
6057,
11,
2685,
3712,
28780,
8,
796,
2922,
62,
3846,
0,
7,
6057,
11,
2685,
13,
312,
87,
8,
198,
198,
37811,
198,
220,
220,
220,
2922,
62,
3846,
0,
7,
6057,
11,
4686,
87,
8,
198,
198,
17563,
2685,
1813,
257,
983,
290,
13690,
35610,
6376,
13,
198,
37811,
198,
8818,
2922,
62,
3846,
0,
7,
6057,
11,
4686,
87,
8,
198,
220,
220,
220,
2685,
796,
983,
13,
46342,
58,
312,
87,
60,
198,
220,
220,
220,
2685,
13,
36955,
3021,
796,
2081,
198,
220,
220,
220,
7766,
62,
9107,
418,
0,
7,
6057,
11,
4686,
87,
8,
198,
220,
220,
220,
983,
13,
3810,
62,
15255,
261,
515,
796,
2685,
13,
10134,
62,
3810,
198,
220,
220,
220,
1441,
2147,
198,
437,
198,
198,
8818,
6056,
62,
3846,
0,
7,
3846,
11,
983,
11,
11774,
3712,
18465,
8,
198,
220,
220,
220,
2685,
13,
2704,
14655,
796,
2081,
198,
220,
220,
220,
983,
13,
1084,
274,
62,
2704,
14655,
15853,
352,
198,
220,
220,
220,
1441,
2147,
198,
437,
198,
198,
8818,
9058,
3419,
198,
220,
220,
220,
44872,
7203,
17563,
27419,
4943,
198,
220,
220,
220,
4953,
796,
2081,
198,
220,
220,
220,
2974,
796,
685,
1238,
11,
1120,
11,
2154,
60,
198,
220,
220,
220,
1312,
796,
657,
198,
220,
220,
220,
981,
4953,
198,
220,
220,
220,
220,
220,
220,
220,
288,
796,
5128,
7203,
16,
25,
2562,
362,
25,
7090,
11,
513,
25,
2408,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
1312,
796,
21136,
7,
5317,
11,
288,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
1312,
18872,
231,
352,
25,
18,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
44872,
7203,
5492,
2922,
352,
11,
362,
11,
393,
513,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4953,
796,
3991,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
3776,
7,
26,
67,
12078,
16193,
1314,
11,
1314,
828,
299,
62,
1084,
274,
28,
46170,
58,
72,
12962,
198,
437,
198,
198,
8818,
711,
3419,
198,
220,
220,
220,
983,
796,
9058,
3419,
198,
220,
220,
220,
711,
7,
6057,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
711,
7,
6057,
8,
198,
198,
11002,
33466,
732,
5723,
287,
45285,
198,
198,
10987,
711,
3419,
284,
779,
351,
4277,
2562,
11,
7090,
290,
2408,
6460,
198,
14478,
983,
2134,
284,
711,
2183,
983,
198,
17469,
8420,
284,
11238,
198,
17469,
5752,
62,
9630,
5721,
62,
9630,
284,
2922,
2685,
357,
68,
13,
70,
13,
352,
362,
8,
198,
37811,
198,
8818,
711,
7,
6057,
8,
198,
220,
220,
220,
2712,
796,
2081,
198,
220,
220,
220,
763,
3669,
796,
6070,
7,
15,
11,
362,
8,
198,
220,
220,
220,
44872,
7,
6057,
8,
198,
220,
220,
220,
981,
2712,
198,
220,
220,
220,
220,
220,
220,
220,
965,
796,
5128,
7203,
19738,
22715,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
965,
6624,
366,
37023,
1,
5633,
357,
7783,
8,
1058,
2147,
198,
220,
220,
220,
220,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
763,
3669,
796,
21136,
12195,
5317,
11,
6626,
7,
2536,
11,
366,
366,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
4929,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4049,
7203,
5492,
1577,
2124,
290,
331,
22715,
304,
13,
70,
13,
513,
362,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
2922,
62,
3846,
0,
7,
6057,
11,
763,
3669,
23029,
198,
220,
220,
220,
220,
220,
220,
220,
611,
983,
13,
3810,
62,
15255,
261,
515,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7766,
7,
6057,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
44872,
7203,
8777,
3827,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
44872,
7203,
366,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
288,
796,
5128,
7203,
13800,
331,
284,
711,
757,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
288,
6624,
366,
88,
1,
5633,
357,
6057,
796,
9058,
28955,
1058,
357,
17916,
796,
3991,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
44872,
7,
6057,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
5128,
7,
16963,
457,
8,
198,
220,
220,
220,
44872,
7,
16963,
457,
8,
198,
220,
220,
220,
1441,
1100,
1370,
3419,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
4296,
0,
7,
6057,
11,
11774,
3712,
18465,
8,
198,
198,
63,
19119,
0,
63,
318,
973,
284,
3359,
983,
1181,
1141,
27785,
13,
198,
63,
19119,
0,
63,
460,
307,
973,
351,
45285,
393,
402,
30488,
25757,
611,
17392,
2884,
1330,
62,
48317,
3419,
198,
37811,
198,
8818,
4296,
0,
7,
6057,
11,
11774,
3712,
18465,
8,
198,
220,
220,
220,
44872,
7,
6057,
8,
198,
437,
198,
198,
37811,
198,
320,
3742,
402,
30488,
3519,
2438,
329,
262,
25757,
198,
37811,
198,
8818,
1330,
62,
48317,
3419,
198,
220,
220,
220,
3108,
796,
2488,
834,
34720,
834,
198,
220,
220,
220,
2291,
7,
6978,
9,
1,
14,
38,
30488,
62,
8205,
72,
13,
20362,
4943,
198,
437,
198
] | 2.216559 | 3,865 |
#!/usr/bin/env julia
using DelimitedFiles
using WaterMeanMeanForce
using ArgParse
s = ArgParseSettings()
s.autofix_names = true
models = join(list_models(), ", ")
integrators = join(list_integrators(), ", ")
@add_arg_table! s begin
"--model"
metavar = "M"
range_tester = in(list_models())
help = "water model ($(models))"
required = true
"--integrator"
metavar = "I"
range_tester = in(list_integrators())
help = "molecular dynamics integrator ($(integrators))"
required = true
"-T"
metavar = "T"
help = "temperature (K)"
arg_type = Float64
required = true
"-R"
metavar = "R"
help = "constraint/restraint distance (nm)"
arg_type = Float64
required = true
"--num-links"
metavar = "P"
help = "number of Trotter links"
arg_type = Int
required = true
"--time-step"
metavar = "T"
help = "time step (ps)"
arg_type = Float64
required = true
"--centroid-friction"
metavar = "G"
help = "centroid friction (1 / ps)"
arg_type = Float64
required = true
"--equil-duration"
metavar = "D"
help = "duration of equilibration (ps)"
arg_type = Float64
required = true
"--umbrella-k"
metavar = "K"
help = "umbrella sampling force constant (kJ / nm^2 mol)"
arg_type = Float64
"--initial-configuration"
metavar = "FILE"
help = "path to initial configuration input file"
"--final-configuration"
metavar = "FILE"
help = "path to final configuration output file"
end
c = parse_args(ARGS, s, as_symbols=true)
if !isnothing(c[:initial_configuration])
qs_init = dropdims(readdlm(c[:initial_configuration]); dims=2)
else
qs_init = nothing
end
mdp_equil = MolecularDynamicsParameters(c[:centroid_friction], c[:time_step],
c[:equil_duration])
if isnothing(c[:umbrella_k])
s = run_constrained_equilibration(get_model(c[:model]),
get_integrator(c[:integrator]), 2, c[:T],
c[:R], c[:num_links], mdp_equil; qs_init)
else
s = run_restrained_equilibration(get_model(c[:model]),
get_integrator(c[:integrator]), 2, c[:T],
c[:R], c[:num_links], mdp_equil,
c[:umbrella_k]; qs_init)
end
if !isnothing(c[:final_configuration])
writedlm(c[:final_configuration], s.qs)
end
| [
2,
48443,
14629,
14,
8800,
14,
24330,
474,
43640,
198,
198,
3500,
4216,
320,
863,
25876,
198,
198,
3500,
5638,
5308,
272,
5308,
272,
10292,
198,
198,
3500,
20559,
10044,
325,
198,
198,
82,
796,
20559,
10044,
325,
26232,
3419,
198,
82,
13,
2306,
1659,
844,
62,
14933,
796,
2081,
198,
198,
27530,
796,
4654,
7,
4868,
62,
27530,
22784,
33172,
366,
8,
198,
18908,
18942,
796,
4654,
7,
4868,
62,
18908,
18942,
22784,
33172,
366,
8,
198,
198,
31,
2860,
62,
853,
62,
11487,
0,
264,
2221,
198,
220,
220,
220,
366,
438,
19849,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1138,
615,
283,
796,
366,
44,
1,
198,
220,
220,
220,
220,
220,
220,
220,
2837,
62,
4879,
353,
796,
287,
7,
4868,
62,
27530,
28955,
198,
220,
220,
220,
220,
220,
220,
220,
1037,
796,
366,
7050,
2746,
7198,
7,
27530,
4008,
1,
198,
220,
220,
220,
220,
220,
220,
220,
2672,
796,
2081,
198,
220,
220,
220,
366,
438,
18908,
12392,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1138,
615,
283,
796,
366,
40,
1,
198,
220,
220,
220,
220,
220,
220,
220,
2837,
62,
4879,
353,
796,
287,
7,
4868,
62,
18908,
18942,
28955,
198,
220,
220,
220,
220,
220,
220,
220,
1037,
796,
366,
76,
2305,
10440,
17262,
4132,
12392,
7198,
7,
18908,
18942,
4008,
1,
198,
220,
220,
220,
220,
220,
220,
220,
2672,
796,
2081,
198,
220,
220,
220,
27444,
51,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1138,
615,
283,
796,
366,
51,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1037,
796,
366,
11498,
21069,
357,
42,
16725,
198,
220,
220,
220,
220,
220,
220,
220,
1822,
62,
4906,
796,
48436,
2414,
198,
220,
220,
220,
220,
220,
220,
220,
2672,
796,
2081,
198,
220,
220,
220,
27444,
49,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1138,
615,
283,
796,
366,
49,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1037,
796,
366,
1102,
2536,
2913,
14,
2118,
16947,
5253,
357,
21533,
16725,
198,
220,
220,
220,
220,
220,
220,
220,
1822,
62,
4906,
796,
48436,
2414,
198,
220,
220,
220,
220,
220,
220,
220,
2672,
796,
2081,
198,
220,
220,
220,
366,
438,
22510,
12,
28751,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1138,
615,
283,
796,
366,
47,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1037,
796,
366,
17618,
286,
8498,
83,
353,
6117,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1822,
62,
4906,
796,
2558,
198,
220,
220,
220,
220,
220,
220,
220,
2672,
796,
2081,
198,
220,
220,
220,
366,
438,
2435,
12,
9662,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1138,
615,
283,
796,
366,
51,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1037,
796,
366,
2435,
2239,
357,
862,
16725,
198,
220,
220,
220,
220,
220,
220,
220,
1822,
62,
4906,
796,
48436,
2414,
198,
220,
220,
220,
220,
220,
220,
220,
2672,
796,
2081,
198,
220,
220,
220,
366,
438,
1087,
3882,
12,
69,
46214,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1138,
615,
283,
796,
366,
38,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1037,
796,
366,
1087,
3882,
23822,
357,
16,
1220,
26692,
16725,
198,
220,
220,
220,
220,
220,
220,
220,
1822,
62,
4906,
796,
48436,
2414,
198,
220,
220,
220,
220,
220,
220,
220,
2672,
796,
2081,
198,
220,
220,
220,
366,
438,
4853,
346,
12,
32257,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1138,
615,
283,
796,
366,
35,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1037,
796,
366,
32257,
286,
1602,
22282,
1358,
357,
862,
16725,
198,
220,
220,
220,
220,
220,
220,
220,
1822,
62,
4906,
796,
48436,
2414,
198,
220,
220,
220,
220,
220,
220,
220,
2672,
796,
2081,
198,
220,
220,
220,
366,
438,
2178,
20481,
12,
74,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1138,
615,
283,
796,
366,
42,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1037,
796,
366,
2178,
20481,
19232,
2700,
6937,
357,
74,
41,
1220,
28642,
61,
17,
18605,
16725,
198,
220,
220,
220,
220,
220,
220,
220,
1822,
62,
4906,
796,
48436,
2414,
198,
220,
220,
220,
366,
438,
36733,
12,
11250,
3924,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1138,
615,
283,
796,
366,
25664,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1037,
796,
366,
6978,
284,
4238,
8398,
5128,
2393,
1,
198,
220,
220,
220,
366,
438,
20311,
12,
11250,
3924,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1138,
615,
283,
796,
366,
25664,
1,
198,
220,
220,
220,
220,
220,
220,
220,
1037,
796,
366,
6978,
284,
2457,
8398,
5072,
2393,
1,
198,
437,
198,
198,
66,
796,
21136,
62,
22046,
7,
1503,
14313,
11,
264,
11,
355,
62,
1837,
2022,
10220,
28,
7942,
8,
198,
198,
361,
5145,
271,
22366,
7,
66,
58,
25,
36733,
62,
11250,
3924,
12962,
198,
220,
220,
220,
10662,
82,
62,
15003,
796,
4268,
67,
12078,
7,
961,
25404,
76,
7,
66,
58,
25,
36733,
62,
11250,
3924,
36563,
5391,
82,
28,
17,
8,
198,
17772,
198,
220,
220,
220,
10662,
82,
62,
15003,
796,
2147,
198,
437,
628,
198,
9132,
79,
62,
4853,
346,
796,
38275,
35,
4989,
873,
48944,
7,
66,
58,
25,
1087,
3882,
62,
69,
46214,
4357,
269,
58,
25,
2435,
62,
9662,
4357,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
58,
25,
4853,
346,
62,
32257,
12962,
198,
198,
361,
318,
22366,
7,
66,
58,
25,
2178,
20481,
62,
74,
12962,
198,
220,
220,
220,
264,
796,
1057,
62,
1102,
2536,
1328,
62,
4853,
22282,
1358,
7,
1136,
62,
19849,
7,
66,
58,
25,
19849,
46570,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
651,
62,
18908,
12392,
7,
66,
58,
25,
18908,
12392,
46570,
362,
11,
269,
58,
25,
51,
4357,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
58,
25,
49,
4357,
269,
58,
25,
22510,
62,
28751,
4357,
285,
26059,
62,
4853,
346,
26,
10662,
82,
62,
15003,
8,
198,
17772,
198,
220,
220,
220,
264,
796,
1057,
62,
2118,
13363,
62,
4853,
22282,
1358,
7,
1136,
62,
19849,
7,
66,
58,
25,
19849,
46570,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
651,
62,
18908,
12392,
7,
66,
58,
25,
18908,
12392,
46570,
362,
11,
269,
58,
25,
51,
4357,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
58,
25,
49,
4357,
269,
58,
25,
22510,
62,
28751,
4357,
285,
26059,
62,
4853,
346,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
58,
25,
2178,
20481,
62,
74,
11208,
10662,
82,
62,
15003,
8,
198,
437,
198,
198,
361,
5145,
271,
22366,
7,
66,
58,
25,
20311,
62,
11250,
3924,
12962,
198,
220,
220,
220,
1991,
276,
75,
76,
7,
66,
58,
25,
20311,
62,
11250,
3924,
4357,
264,
13,
48382,
8,
198,
437,
198
] | 1.974589 | 1,338 |
# This is code for computing a transit model and derivatives integrated over
# a time step, giving the fluence in units of time (since
# flux is normalized to unity).
using Cubature
include("transit_poly_struct.jl")
# Now the version with derivatives:
function integrate_timestep_gradient!(param::Array{T,1},trans::Transit_Struct{T},t1::T,t2::T,tol::T,maxdepth::Int64) where {T <: Real}
function fill_flux!(tmid::T,fmid0::T,trans_mid::Transit_Struct{T}) where {T <: Real}
fmid = Array{T}(6+trans_mid.n)
fmid[1] = fmid0 # flux
fmid[2] = trans_mid.dfdrb[1] # r derivative
fmid[3] = trans_mid.dfdrb[2]/trans_mid.b*param[2]^2*(param[1]-tmid) # t0 derivative
fmid[4] = trans_mid.dfdrb[2]*param[2]/trans_mid.b*(tmid-param[1])^2 # v derivative
fmid[5] = trans_mid.dfdrb[2]*param[3]/trans_mid.b # b0 derivative
@inbounds for i=1:trans_mid.n+1
fmid[5+i] = trans_mid.dfdg[i] # d_i derivatives
end
return fmid
end
function solver(time::T) where {T <: Real}
# This predicts the impact parameter as a function of time in
# terms of the transit_struct.
# For now, transit is approximated as a straight line, where params are given as:
# param[1] = t0 (mid-transit time; units of days/JD)
# param[2] = v/R_* (sky velocity in terms of radius of the star - units are day^{-1}
# param[3] = b (impact parameter at time t0)
return sqrt(param[3]^2+(param[2]*(time-param[1]))^2)
end
# Function to define the vector integration in cubature:
function transit_flux_derivative(tmid::T,fmid::Array{T,1}) where {T <: Real}
trans.b = solver(tmid)
fmid0 = transit_poly_d!(trans)
fmid .= fill_flux!(tmid,fmid0,trans)
# println("b: ",trans.b," f/df: ",fmid)
return
end
fint,ferr = hquadrature(trans.n+6,transit_flux_derivative,t1,t2,abstol=tol)
return fint
end
| [
2,
770,
318,
2438,
329,
14492,
257,
11168,
2746,
290,
28486,
11521,
625,
198,
2,
257,
640,
2239,
11,
3501,
262,
6562,
594,
287,
4991,
286,
640,
357,
20777,
220,
198,
2,
28462,
318,
39279,
284,
14111,
737,
198,
198,
3500,
7070,
1300,
198,
198,
17256,
7203,
7645,
270,
62,
35428,
62,
7249,
13,
20362,
4943,
198,
198,
2,
2735,
262,
2196,
351,
28486,
25,
198,
8818,
19386,
62,
16514,
395,
538,
62,
49607,
0,
7,
17143,
3712,
19182,
90,
51,
11,
16,
5512,
7645,
3712,
8291,
270,
62,
44909,
90,
51,
5512,
83,
16,
3712,
51,
11,
83,
17,
3712,
51,
11,
83,
349,
3712,
51,
11,
9806,
18053,
3712,
5317,
2414,
8,
810,
1391,
51,
1279,
25,
6416,
92,
628,
220,
2163,
6070,
62,
69,
22564,
0,
7,
83,
13602,
3712,
51,
11,
69,
13602,
15,
3712,
51,
11,
7645,
62,
13602,
3712,
8291,
270,
62,
44909,
90,
51,
30072,
810,
1391,
51,
1279,
25,
6416,
92,
198,
220,
277,
13602,
796,
15690,
90,
51,
92,
7,
21,
10,
7645,
62,
13602,
13,
77,
8,
198,
220,
277,
13602,
58,
16,
60,
796,
277,
13602,
15,
220,
220,
1303,
28462,
198,
220,
277,
13602,
58,
17,
60,
796,
1007,
62,
13602,
13,
7568,
7109,
65,
58,
16,
60,
220,
1303,
374,
27255,
198,
220,
277,
13602,
58,
18,
60,
796,
1007,
62,
13602,
13,
7568,
7109,
65,
58,
17,
60,
14,
7645,
62,
13602,
13,
65,
9,
17143,
58,
17,
60,
61,
17,
9,
7,
17143,
58,
16,
45297,
83,
13602,
8,
220,
1303,
256,
15,
27255,
198,
220,
277,
13602,
58,
19,
60,
796,
1007,
62,
13602,
13,
7568,
7109,
65,
58,
17,
60,
9,
17143,
58,
17,
60,
14,
7645,
62,
13602,
13,
65,
9,
7,
83,
13602,
12,
17143,
58,
16,
12962,
61,
17,
220,
1303,
410,
27255,
198,
220,
277,
13602,
58,
20,
60,
796,
1007,
62,
13602,
13,
7568,
7109,
65,
58,
17,
60,
9,
17143,
58,
18,
60,
14,
7645,
62,
13602,
13,
65,
220,
1303,
275,
15,
27255,
198,
220,
2488,
259,
65,
3733,
329,
1312,
28,
16,
25,
7645,
62,
13602,
13,
77,
10,
16,
198,
220,
220,
220,
277,
13602,
58,
20,
10,
72,
60,
796,
1007,
62,
13602,
13,
7568,
67,
70,
58,
72,
60,
220,
1303,
288,
62,
72,
28486,
198,
220,
886,
198,
220,
1441,
277,
13602,
198,
220,
886,
628,
220,
2163,
1540,
332,
7,
2435,
3712,
51,
8,
810,
1391,
51,
1279,
25,
6416,
92,
198,
220,
1303,
770,
26334,
262,
2928,
11507,
355,
257,
2163,
286,
640,
287,
198,
220,
1303,
2846,
286,
262,
11168,
62,
7249,
13,
198,
220,
1303,
1114,
783,
11,
11168,
318,
5561,
15655,
355,
257,
3892,
1627,
11,
810,
42287,
389,
1813,
355,
25,
198,
220,
1303,
5772,
58,
16,
60,
796,
256,
15,
357,
13602,
12,
7645,
270,
640,
26,
4991,
286,
1528,
14,
37882,
8,
198,
220,
1303,
5772,
58,
17,
60,
796,
410,
14,
49,
62,
9,
357,
15688,
15432,
287,
2846,
286,
16874,
286,
262,
3491,
532,
4991,
389,
1110,
36796,
12,
16,
92,
198,
220,
1303,
5772,
58,
18,
60,
796,
275,
357,
48240,
11507,
379,
640,
256,
15,
8,
198,
220,
1441,
19862,
17034,
7,
17143,
58,
18,
60,
61,
17,
33747,
17143,
58,
17,
60,
9,
7,
2435,
12,
17143,
58,
16,
60,
4008,
61,
17,
8,
198,
220,
886,
628,
220,
1303,
15553,
284,
8160,
262,
15879,
11812,
287,
13617,
1300,
25,
198,
220,
2163,
11168,
62,
69,
22564,
62,
1082,
452,
876,
7,
83,
13602,
3712,
51,
11,
69,
13602,
3712,
19182,
90,
51,
11,
16,
30072,
810,
1391,
51,
1279,
25,
6416,
92,
198,
220,
1007,
13,
65,
796,
1540,
332,
7,
83,
13602,
8,
198,
220,
277,
13602,
15,
796,
11168,
62,
35428,
62,
67,
0,
7,
7645,
8,
198,
220,
277,
13602,
764,
28,
6070,
62,
69,
22564,
0,
7,
83,
13602,
11,
69,
13602,
15,
11,
7645,
8,
198,
2,
220,
44872,
7203,
65,
25,
33172,
7645,
13,
65,
553,
277,
14,
7568,
25,
33172,
69,
13602,
8,
198,
220,
1441,
198,
220,
886,
198,
220,
220,
198,
220,
277,
600,
11,
2232,
81,
796,
289,
421,
41909,
1300,
7,
7645,
13,
77,
10,
21,
11,
7645,
270,
62,
69,
22564,
62,
1082,
452,
876,
11,
83,
16,
11,
83,
17,
11,
397,
301,
349,
28,
83,
349,
8,
198,
7783,
277,
600,
198,
437,
198
] | 2.471956 | 731 |
function update_gam!(i::Int, n::Int, j::Int, s::State, c::Constants, d::Data)
k = s.lam[i][n]
if k > 0
z = s.Z[j, k]
logpriorVec = log.(s.eta[z][i, j, :])
loglikeVec = logpdf.(Normal.(mus(z, s, c, d), sqrt(s.sig2[i])), s.y_imputed[i][n, j])
logPostVec = logpriorVec .+ loglikeVec
s.gam[i][n, j] = MCMC.wsample_logprob(logPostVec)
else
s.gam[i][n, j] = 0
end
end
function update_gam!(s::State, c::Constants, d::Data)
for i in 1:d.I
for j in 1:d.J
for n in 1:d.N[i]
update_gam!(i, n, j, s, c, d)
end
end
end
end
| [
8818,
4296,
62,
28483,
0,
7,
72,
3712,
5317,
11,
299,
3712,
5317,
11,
474,
3712,
5317,
11,
264,
3712,
9012,
11,
269,
3712,
34184,
1187,
11,
288,
3712,
6601,
8,
198,
220,
479,
796,
264,
13,
2543,
58,
72,
7131,
77,
60,
198,
220,
611,
479,
1875,
657,
198,
220,
220,
220,
1976,
796,
264,
13,
57,
58,
73,
11,
479,
60,
198,
220,
220,
220,
2604,
3448,
273,
53,
721,
796,
2604,
12195,
82,
13,
17167,
58,
89,
7131,
72,
11,
474,
11,
1058,
12962,
198,
220,
220,
220,
2604,
2339,
53,
721,
796,
2604,
12315,
12195,
26447,
12195,
14664,
7,
89,
11,
264,
11,
269,
11,
288,
828,
19862,
17034,
7,
82,
13,
82,
328,
17,
58,
72,
12962,
828,
264,
13,
88,
62,
320,
17128,
58,
72,
7131,
77,
11,
474,
12962,
198,
220,
220,
220,
2604,
6307,
53,
721,
796,
2604,
3448,
273,
53,
721,
764,
10,
2604,
2339,
53,
721,
198,
220,
220,
220,
264,
13,
28483,
58,
72,
7131,
77,
11,
474,
60,
796,
13122,
9655,
13,
18504,
1403,
62,
6404,
1676,
65,
7,
6404,
6307,
53,
721,
8,
198,
220,
2073,
198,
220,
220,
220,
264,
13,
28483,
58,
72,
7131,
77,
11,
474,
60,
796,
657,
198,
220,
886,
198,
437,
198,
198,
8818,
4296,
62,
28483,
0,
7,
82,
3712,
9012,
11,
269,
3712,
34184,
1187,
11,
288,
3712,
6601,
8,
198,
220,
329,
1312,
287,
352,
25,
67,
13,
40,
198,
220,
220,
220,
329,
474,
287,
352,
25,
67,
13,
41,
198,
220,
220,
220,
220,
220,
329,
299,
287,
352,
25,
67,
13,
45,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
4296,
62,
28483,
0,
7,
72,
11,
299,
11,
474,
11,
264,
11,
269,
11,
288,
8,
198,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
886,
198,
437,
628
] | 1.840256 | 313 |
using Documenter, Jets, LinearAlgebra, Random
makedocs(
sitename="Jets.jl",
modules=[Jets],
pages = [ "index.md", "reference.md" ]
)
deploydocs(
repo = "github.com/ChevronETC/Jets.jl.git"
)
| [
3500,
16854,
263,
11,
14728,
11,
44800,
2348,
29230,
11,
14534,
198,
198,
76,
4335,
420,
82,
7,
198,
220,
220,
220,
1650,
12453,
2625,
41,
1039,
13,
20362,
1600,
198,
220,
220,
220,
13103,
41888,
41,
1039,
4357,
198,
220,
220,
220,
5468,
796,
685,
366,
9630,
13,
9132,
1600,
366,
35790,
13,
9132,
1,
2361,
198,
8,
198,
198,
2934,
1420,
31628,
7,
198,
220,
220,
220,
29924,
796,
366,
12567,
13,
785,
14,
7376,
85,
1313,
2767,
34,
14,
41,
1039,
13,
20362,
13,
18300,
1,
198,
8,
198
] | 2.26087 | 92 |
module test_stdlib_statistics
using Statistics, Test
X = rand(5)
@test cor(X, 2X) == 1.0
@test cov(X, 2X) - cov(X, X) == var(X)
end # module test_stdlib_statistics
| [
21412,
1332,
62,
19282,
8019,
62,
14269,
3969,
198,
198,
3500,
14370,
11,
6208,
198,
198,
55,
796,
43720,
7,
20,
8,
198,
198,
31,
9288,
1162,
7,
55,
11,
362,
55,
8,
6624,
352,
13,
15,
198,
31,
9288,
39849,
7,
55,
11,
362,
55,
8,
532,
39849,
7,
55,
11,
1395,
8,
6624,
1401,
7,
55,
8,
198,
198,
437,
1303,
8265,
1332,
62,
19282,
8019,
62,
14269,
3969,
198
] | 2.333333 | 72 |
# Dispatchable and non-dispatchable generators
## Expressions
"Curtailed power of a non-dispatchable generator as the difference between its reference power and the generated power."
function expression_gen_curtailment(pm::_PM.AbstractPowerModel; nw::Int=_PM.nw_id_default, report::Bool=true)
pgcurt = _PM.var(pm, nw)[:pgcurt] = Dict{Int,Any}(
i => ndgen["pmax"] - _PM.var(pm,nw,:pg,i) for (i,ndgen) in _PM.ref(pm,nw,:ndgen)
)
if report
_IM.sol_component_fixed(pm, _PM.pm_it_sym, nw, :gen, :pgcurt, _PM.ids(pm, nw, :dgen), 0.0)
_PM.sol_component_value(pm, nw, :gen, :pgcurt, _PM.ids(pm, nw, :ndgen), pgcurt)
end
end
| [
2,
35934,
540,
290,
1729,
12,
6381,
17147,
540,
27298,
628,
198,
2235,
10604,
507,
198,
198,
1,
34,
3325,
6255,
1176,
286,
257,
1729,
12,
6381,
17147,
540,
17301,
355,
262,
3580,
1022,
663,
4941,
1176,
290,
262,
7560,
1176,
526,
198,
8818,
5408,
62,
5235,
62,
66,
3325,
603,
434,
7,
4426,
3712,
62,
5868,
13,
23839,
13434,
17633,
26,
299,
86,
3712,
5317,
28,
62,
5868,
13,
47516,
62,
312,
62,
12286,
11,
989,
3712,
33,
970,
28,
7942,
8,
198,
220,
220,
220,
23241,
66,
3325,
796,
4808,
5868,
13,
7785,
7,
4426,
11,
299,
86,
38381,
25,
6024,
66,
3325,
60,
796,
360,
713,
90,
5317,
11,
7149,
92,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1312,
5218,
299,
67,
5235,
14692,
4426,
897,
8973,
532,
4808,
5868,
13,
7785,
7,
4426,
11,
47516,
11,
25,
6024,
11,
72,
8,
329,
357,
72,
11,
358,
5235,
8,
287,
4808,
5868,
13,
5420,
7,
4426,
11,
47516,
11,
25,
358,
5235,
8,
198,
220,
220,
220,
1267,
198,
220,
220,
220,
611,
989,
198,
220,
220,
220,
220,
220,
220,
220,
4808,
3955,
13,
34453,
62,
42895,
62,
34021,
7,
4426,
11,
4808,
5868,
13,
4426,
62,
270,
62,
37047,
11,
299,
86,
11,
1058,
5235,
11,
1058,
6024,
66,
3325,
11,
4808,
5868,
13,
2340,
7,
4426,
11,
299,
86,
11,
1058,
67,
5235,
828,
657,
13,
15,
8,
198,
220,
220,
220,
220,
220,
220,
220,
4808,
5868,
13,
34453,
62,
42895,
62,
8367,
7,
4426,
11,
299,
86,
11,
1058,
5235,
11,
1058,
6024,
66,
3325,
11,
4808,
5868,
13,
2340,
7,
4426,
11,
299,
86,
11,
1058,
358,
5235,
828,
23241,
66,
3325,
8,
198,
220,
220,
220,
886,
198,
437,
198
] | 2.256849 | 292 |
# ---
# title: 1545. Find Kth Bit in Nth Binary String
# id: problem1545
# author: Tian Jun
# date: 2020-10-31
# difficulty: Medium
# categories: String
# link: <https://leetcode.com/problems/find-kth-bit-in-nth-binary-string/description/>
# hidden: true
# ---
#
# Given two positive integers `n` and `k`, the binary string `Sn` is formed as
# follows:
#
# * `S1 = "0"`
# * `Si = Si-1 + "1" + reverse(invert(Si-1))` for `i > 1`
#
# Where `+` denotes the concatenation operation, `reverse(x)` returns the
# reversed string x, and `invert(x)` inverts all the bits in x (0 changes to 1
# and 1 changes to 0).
#
# For example, the first 4 strings in the above sequence are:
#
# * `S1 = "0"`
# * `S2 = "0 **1** 1"`
# * `S3 = "011 **1** 001"`
# * `S4 = "0111001 **1** 0110001"`
#
# Return _the_ `kth` _bit_ _in_ `Sn`. It is guaranteed that `k` is valid for
# the given `n`.
#
#
#
# **Example 1:**
#
#
#
# Input: n = 3, k = 1
# Output: "0"
# Explanation: S3 is " ** _0_** 111001". The first bit is "0".
#
#
# **Example 2:**
#
#
#
# Input: n = 4, k = 11
# Output: "1"
# Explanation: S4 is "0111001101 ** _1_** 0001". The 11th bit is "1".
#
#
# **Example 3:**
#
#
#
# Input: n = 1, k = 1
# Output: "0"
#
#
# **Example 4:**
#
#
#
# Input: n = 2, k = 3
# Output: "1"
#
#
#
#
# **Constraints:**
#
# * `1 <= n <= 20`
# * `1 <= k <= 2n - 1`
#
#
## @lc code=start
using LeetCode
## add your code here:
## @lc code=end
| [
2,
11420,
198,
2,
3670,
25,
1315,
2231,
13,
9938,
509,
400,
4722,
287,
399,
400,
45755,
10903,
198,
2,
4686,
25,
1917,
1314,
2231,
198,
2,
1772,
25,
20834,
7653,
198,
2,
3128,
25,
12131,
12,
940,
12,
3132,
198,
2,
8722,
25,
13398,
198,
2,
9376,
25,
10903,
198,
2,
2792,
25,
1279,
5450,
1378,
293,
316,
8189,
13,
785,
14,
1676,
22143,
14,
19796,
12,
74,
400,
12,
2545,
12,
259,
12,
77,
400,
12,
39491,
12,
8841,
14,
11213,
15913,
198,
2,
7104,
25,
2081,
198,
2,
11420,
198,
2,
220,
198,
2,
11259,
734,
3967,
37014,
4600,
77,
63,
290,
4600,
74,
47671,
262,
13934,
4731,
220,
4600,
16501,
63,
318,
7042,
355,
198,
2,
5679,
25,
198,
2,
220,
198,
2,
220,
220,
1635,
4600,
50,
16,
796,
366,
15,
1,
63,
198,
2,
220,
220,
1635,
4600,
42801,
796,
15638,
12,
16,
1343,
366,
16,
1,
1343,
9575,
7,
259,
1851,
7,
42801,
12,
16,
4008,
63,
329,
4600,
72,
1875,
352,
63,
198,
2,
220,
198,
2,
6350,
4600,
10,
63,
43397,
262,
1673,
36686,
341,
4905,
11,
4600,
50188,
7,
87,
8,
63,
5860,
262,
198,
2,
17687,
4731,
2124,
11,
290,
4600,
259,
1851,
7,
87,
8,
63,
287,
24040,
477,
262,
10340,
287,
2124,
357,
15,
2458,
284,
352,
198,
2,
290,
352,
2458,
284,
657,
737,
198,
2,
220,
198,
2,
1114,
1672,
11,
262,
717,
604,
13042,
287,
262,
2029,
8379,
389,
25,
198,
2,
220,
198,
2,
220,
220,
1635,
4600,
50,
16,
796,
366,
15,
1,
63,
198,
2,
220,
220,
1635,
4600,
50,
17,
796,
366,
15,
12429,
16,
1174,
352,
1,
63,
198,
2,
220,
220,
1635,
4600,
50,
18,
796,
366,
28555,
12429,
16,
1174,
3571,
16,
1,
63,
198,
2,
220,
220,
1635,
4600,
50,
19,
796,
366,
486,
1157,
8298,
12429,
16,
1174,
5534,
3064,
486,
1,
63,
198,
2,
220,
198,
2,
8229,
4808,
1169,
62,
4600,
74,
400,
63,
4808,
2545,
62,
4808,
259,
62,
220,
4600,
16501,
44646,
632,
318,
11462,
326,
4600,
74,
63,
318,
4938,
329,
198,
2,
262,
1813,
4600,
77,
44646,
198,
2,
220,
198,
2,
220,
198,
2,
220,
198,
2,
12429,
16281,
352,
25,
1174,
198,
2,
220,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
220,
220,
220,
23412,
25,
299,
796,
513,
11,
479,
796,
352,
198,
2,
220,
220,
220,
220,
25235,
25,
366,
15,
1,
198,
2,
220,
220,
220,
220,
50125,
341,
25,
311,
18,
318,
366,
12429,
4808,
15,
62,
1174,
13374,
8298,
1911,
383,
717,
1643,
318,
366,
15,
1911,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
198,
2,
12429,
16281,
362,
25,
1174,
198,
2,
220,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
220,
220,
220,
23412,
25,
299,
796,
604,
11,
479,
796,
1367,
198,
2,
220,
220,
220,
220,
25235,
25,
366,
16,
1,
198,
2,
220,
220,
220,
220,
50125,
341,
25,
311,
19,
318,
366,
486,
42060,
1157,
486,
12429,
4808,
16,
62,
1174,
3571,
486,
1911,
383,
1367,
400,
1643,
318,
366,
16,
1911,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
198,
2,
12429,
16281,
513,
25,
1174,
198,
2,
220,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
220,
220,
220,
23412,
25,
299,
796,
352,
11,
479,
796,
352,
198,
2,
220,
220,
220,
220,
25235,
25,
366,
15,
1,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
198,
2,
12429,
16281,
604,
25,
1174,
198,
2,
220,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
220,
220,
220,
23412,
25,
299,
796,
362,
11,
479,
796,
513,
198,
2,
220,
220,
220,
220,
25235,
25,
366,
16,
1,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
198,
2,
220,
198,
2,
220,
198,
2,
12429,
3103,
2536,
6003,
25,
1174,
198,
2,
220,
198,
2,
220,
220,
1635,
4600,
16,
19841,
299,
19841,
1160,
63,
198,
2,
220,
220,
1635,
4600,
16,
19841,
479,
19841,
362,
77,
532,
352,
63,
198,
2,
220,
198,
2,
220,
198,
2235,
2488,
44601,
2438,
28,
9688,
198,
3500,
1004,
316,
10669,
198,
198,
2235,
751,
534,
2438,
994,
25,
198,
2235,
2488,
44601,
2438,
28,
437,
198
] | 2.069705 | 746 |
"""copy a FEFunction on a FE Space"""
clone_fe_function(space,f)=FEFunction(space,copy(get_free_dof_values(f)))
"""
Given an arbitrary number of FEFunction arguments,
returns a tuple with their corresponding free DoF Values
"""
function Gridap.get_free_dof_values(functions...)
map(get_free_dof_values,functions)
end
function setup_mixed_spaces(model, order)
reffe_rt = ReferenceFE(raviart_thomas, Float64, order)
V = FESpace(model, reffe_rt ; conformity=:HDiv)
U = TrialFESpace(V)
reffe_lgn = ReferenceFE(lagrangian, Float64, order)
Q = FESpace(model, reffe_lgn; conformity=:L2)
P = TrialFESpace(Q)
reffe_lgn = ReferenceFE(lagrangian, Float64, order+1)
S = FESpace(model, reffe_lgn; conformity=:H1)
R = TrialFESpace(S)
R, S, U, V, P, Q
end
function setup_and_factorize_mass_matrices(dΩ,
R, S, U, V, P, Q;
mass_matrix_solver=BackslashSolver())
amm(a,b) = ∫(a⋅b)dΩ
H1MM = assemble_matrix(amm, R, S)
RTMM = assemble_matrix(amm, U, V)
L2MM = assemble_matrix(amm, P, Q)
ssH1MM = symbolic_setup(mass_matrix_solver,H1MM)
nsH1MM = numerical_setup(ssH1MM,H1MM)
ssRTMM = symbolic_setup(mass_matrix_solver,RTMM)
nsRTMM = numerical_setup(ssRTMM,RTMM)
ssL2MM = symbolic_setup(mass_matrix_solver,L2MM)
nsL2MM = numerical_setup(ssL2MM,L2MM)
H1MM, RTMM, L2MM, nsH1MM, nsRTMM, nsL2MM
end
function new_vtk_step(Ω,file,_cellfields)
n = size(_cellfields)[1]
createvtk(Ω,
file,
cellfields=_cellfields,
nsubcells=n)
end
| [
37811,
30073,
257,
18630,
22203,
319,
257,
18630,
4687,
37811,
198,
21018,
62,
5036,
62,
8818,
7,
13200,
11,
69,
47505,
15112,
22203,
7,
13200,
11,
30073,
7,
1136,
62,
5787,
62,
67,
1659,
62,
27160,
7,
69,
22305,
198,
198,
37811,
198,
11259,
281,
14977,
1271,
286,
18630,
22203,
7159,
11,
198,
5860,
257,
46545,
351,
511,
11188,
1479,
2141,
37,
27068,
198,
37811,
198,
8818,
24846,
499,
13,
1136,
62,
5787,
62,
67,
1659,
62,
27160,
7,
12543,
2733,
23029,
198,
220,
3975,
7,
1136,
62,
5787,
62,
67,
1659,
62,
27160,
11,
12543,
2733,
8,
198,
437,
198,
198,
8818,
9058,
62,
76,
2966,
62,
2777,
2114,
7,
19849,
11,
1502,
8,
198,
220,
302,
16658,
62,
17034,
220,
796,
20984,
15112,
7,
4108,
72,
433,
62,
400,
16911,
11,
48436,
2414,
11,
1502,
8,
198,
220,
569,
796,
376,
1546,
10223,
7,
19849,
11,
302,
16658,
62,
17034,
2162,
39974,
28,
25,
10227,
452,
8,
198,
220,
471,
796,
21960,
37,
1546,
10223,
7,
53,
8,
198,
220,
302,
16658,
62,
75,
4593,
796,
20984,
15112,
7,
30909,
36985,
666,
11,
48436,
2414,
11,
1502,
8,
198,
220,
1195,
796,
376,
1546,
10223,
7,
19849,
11,
302,
16658,
62,
75,
4593,
26,
39974,
28,
25,
43,
17,
8,
198,
220,
350,
796,
21960,
37,
1546,
10223,
7,
48,
8,
198,
220,
302,
16658,
62,
75,
4593,
796,
20984,
15112,
7,
30909,
36985,
666,
11,
48436,
2414,
11,
1502,
10,
16,
8,
198,
220,
311,
796,
376,
1546,
10223,
7,
19849,
11,
302,
16658,
62,
75,
4593,
26,
39974,
28,
25,
39,
16,
8,
198,
220,
371,
796,
21960,
37,
1546,
10223,
7,
50,
8,
628,
220,
371,
11,
311,
11,
471,
11,
569,
11,
350,
11,
1195,
198,
437,
198,
198,
8818,
9058,
62,
392,
62,
31412,
1096,
62,
22208,
62,
6759,
45977,
7,
67,
138,
102,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
371,
11,
311,
11,
471,
11,
569,
11,
350,
11,
1195,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2347,
62,
6759,
8609,
62,
82,
14375,
28,
33,
4595,
17055,
50,
14375,
28955,
198,
220,
716,
76,
7,
64,
11,
65,
8,
796,
18872,
104,
7,
64,
158,
233,
227,
65,
8,
67,
138,
102,
198,
220,
367,
16,
12038,
220,
220,
796,
25432,
62,
6759,
8609,
7,
6475,
11,
371,
11,
311,
8,
198,
220,
11923,
12038,
220,
220,
796,
25432,
62,
6759,
8609,
7,
6475,
11,
471,
11,
569,
8,
198,
220,
406,
17,
12038,
220,
220,
796,
25432,
62,
6759,
8609,
7,
6475,
11,
350,
11,
1195,
8,
628,
220,
37786,
39,
16,
12038,
796,
18975,
62,
40406,
7,
22208,
62,
6759,
8609,
62,
82,
14375,
11,
39,
16,
12038,
8,
198,
220,
36545,
39,
16,
12038,
796,
29052,
62,
40406,
7,
824,
39,
16,
12038,
11,
39,
16,
12038,
8,
628,
220,
37786,
14181,
12038,
796,
18975,
62,
40406,
7,
22208,
62,
6759,
8609,
62,
82,
14375,
11,
14181,
12038,
8,
198,
220,
36545,
14181,
12038,
796,
29052,
62,
40406,
7,
824,
14181,
12038,
11,
14181,
12038,
8,
628,
220,
37786,
43,
17,
12038,
796,
18975,
62,
40406,
7,
22208,
62,
6759,
8609,
62,
82,
14375,
11,
43,
17,
12038,
8,
198,
220,
36545,
43,
17,
12038,
796,
29052,
62,
40406,
7,
824,
43,
17,
12038,
11,
43,
17,
12038,
8,
628,
220,
367,
16,
12038,
11,
11923,
12038,
11,
406,
17,
12038,
11,
36545,
39,
16,
12038,
11,
36545,
14181,
12038,
11,
36545,
43,
17,
12038,
198,
437,
198,
198,
8818,
649,
62,
85,
30488,
62,
9662,
7,
138,
102,
11,
7753,
11,
62,
3846,
25747,
8,
198,
220,
299,
796,
2546,
28264,
3846,
25747,
38381,
16,
60,
198,
220,
2251,
85,
30488,
7,
138,
102,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2393,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2685,
25747,
28,
62,
3846,
25747,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
299,
7266,
46342,
28,
77,
8,
198,
437,
198
] | 2.124498 | 747 |
using GenomicAnnotations
using BioSequences
using Test
@testset "GenomicAnnotations" begin
@testset "GenBank parsing" begin
s = " gene 1..1"
@test GenBank.parseposition(s) == (:gene, Locus(1:1, '+'))
s = " gene complement(order(3300..4037,4047..4052))"
@test GenBank.parseposition(s) == (:gene, Locus(3300:4052, '-', true, true, UnitRange{Int}[3300:4037, 4047:4052], false))
chrs = collect(open(GenBank.Reader, "example.gbk"))
@test length(chrs) == 2
@test chrs[2].name == "plasmid1"
end
chr = collect(open(GenBank.Reader, "example.gbk"))[1]
@testset "GFF parsing" begin
open(GFF.Writer, "example.gff") do w
write(w, chr)
end
gff = collect(open(GFF.Reader, "example.gff"))[1]
@test begin
gbkbuf = IOBuffer()
gffbuf = IOBuffer()
print(gbkbuf, chr.genes[2:4])
print(gffbuf, gff.genes[2:4])
String(take!(gbkbuf)) == String(take!(gffbuf))
end
end
@testset "Extended methods" begin
@test length(chr.genes[1]) == length(sequence(chr.genes[1]))
@test length(chr.sequence) == length(sequence(chr))
end
@testset "Gene properties" begin
@test length(propertynames(chr.genes[1])) == 12
@test chr.genes[2].locus_tag == "tag01"
@test (chr.genes[2].locus_tag = "tag01") == "tag01"
@test begin
chr.genes[2].test = "New column"
chr.genes[2].test == "New column" && all(ismissing, chr.genes[[1,3,4,5,6]].test)
end
@test get(chr.genes[1], :locus_tag, "") == ""
@test get(chr.genes[2], :locus_tag, "") == "tag01"
@test get(chr.genes[2], :locustag, "") == ""
@test begin
GenomicAnnotations.pushproperty!(chr.genes[2], :db_xref, "GI:123")
chr.genes[2].db_xref == ["GI:1293614", "GI:123"]
end
@test GenomicAnnotations.vectorise(Union{Missing,Int}[1,1,1]) == [[1],[1],[1]]
end
@testset "Iteration" begin
@test length([g.locus_tag for g in chr.genes]) == 7
end
@testset "Adding/removing genes" begin
addgene!(chr, :CDS, Locus(300:390, '+'), locus_tag = "tag04")
@test chr.genes[end].locus_tag == "tag04"
delete!(chr.genes[end])
@test chr.genes[end].locus_tag == "reg01"
end
@testset "@genes" begin
@test length(union(@genes(chr, CDS), @genes(chr, !CDS))) == length(chr.genes)
@test length(intersect(@genes(chr, CDS), @genes(chr, !CDS))) == 0
@test length(union(@genes(chr, gene), @genes(chr, !gene))) == length(chr.genes)
@test length(intersect(@genes(chr, gene), @genes(chr, !gene))) == 0
@test length(@genes(chr)) == length(chr.genes)
@test @genes(chr, feature(gene) == $:CDS) == chr.genes[[2,4,6]]
@test @genes(chr, feature(gene) == $:CDS) == @genes(chr, CDS)
@test @genes(chr, iscomplement(gene)) == chr.genes[[5,6,7]]
@test @genes(chr, feature(gene) == $:CDS, !iscomplement(gene)) == chr.genes[[2,4]]
@test @genes(chr, length(gene) < 300)[1] == chr.genes[2]
@test length(@genes(chr, get(gene, :locus_tag, "") == "")) == 3
gene = chr.genes[3]
@test @genes(chr, gene == $gene)[1] == chr.genes[3]
d = Dict(:a => "tag01")
@test @genes(chr, :locus_tag == d[$:a]) == @genes(chr, :locus_tag == "tag01")
end
@testset "Broadcast" begin
# Broadcasted assignment on existing property
chr.genes[3:4].gene .= "AXL2P"
@test all(chr.genes[3:4].gene .== "AXL2P")
# Broadcasted assignment on previously missing property
chr.genes[3:4].newproperty .= true
@test all(chr.genes[3:4].newproperty .== true)
# Broadcasted assignment with @genes
@genes(chr, gene).newproperty .= false
@test all(chr.genes[[3,5]].newproperty .== false)
end
@testset "Locus" begin
loc = Locus(1:1, '.', true, true, UnitRange{Int}[], false)
@test Locus() == loc
@test locus(chr.genes[2]) < locus(chr.genes[4])
@test locus(chr.genes[2]) == locus(chr.genes[2])
@test iscomplement(chr.genes[2]) == false
@test iscomplement(chr.genes[5]) == true
end
seq = dna"atgtccatatacaacggtatctccacctcaggtttagatctcaacaacggaaccattgccgacatgagacagttaggtatcgtcgagagttacaagctaaaacgagcagtagtcagctctgcatctgaagccgctgaagttctactaagggtggataacatcatccgtgcaagaccaagaaccgccaatagacaacatatgtaa"
@test sequence(chr.genes[2]) == seq
@test length(chr.genes[2]) == length(seq)
@testset "Empty Record" begin
chr = GenBank.Record()
@test chr.name == ""
@test chr.sequence == dna""
@test chr.header == ""
@test chr.genes == Gene[]
@test names(chr.genedata) == []
end
end
| [
3500,
5215,
10179,
2025,
30078,
198,
3500,
16024,
44015,
3007,
198,
3500,
6208,
198,
198,
31,
9288,
2617,
366,
13746,
10179,
2025,
30078,
1,
2221,
198,
220,
220,
220,
2488,
9288,
2617,
366,
13746,
28650,
32096,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
264,
796,
366,
220,
220,
220,
220,
9779,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
352,
492,
16,
1,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
5215,
28650,
13,
29572,
9150,
7,
82,
8,
6624,
357,
25,
70,
1734,
11,
406,
10901,
7,
16,
25,
16,
11,
705,
10,
6,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
264,
796,
366,
220,
220,
220,
220,
9779,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
16829,
7,
2875,
7,
2091,
405,
492,
1821,
2718,
11,
1821,
2857,
492,
1821,
4309,
4008,
1,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
5215,
28650,
13,
29572,
9150,
7,
82,
8,
6624,
357,
25,
70,
1734,
11,
406,
10901,
7,
2091,
405,
25,
1821,
4309,
11,
705,
12,
3256,
2081,
11,
2081,
11,
11801,
17257,
90,
5317,
92,
58,
2091,
405,
25,
1821,
2718,
11,
2319,
2857,
25,
1821,
4309,
4357,
3991,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
442,
3808,
796,
2824,
7,
9654,
7,
13746,
28650,
13,
33634,
11,
366,
20688,
13,
22296,
74,
48774,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
4129,
7,
354,
3808,
8,
6624,
362,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
442,
3808,
58,
17,
4083,
3672,
6624,
366,
489,
8597,
312,
16,
1,
198,
220,
220,
220,
886,
628,
220,
220,
220,
442,
81,
796,
2824,
7,
9654,
7,
13746,
28650,
13,
33634,
11,
366,
20688,
13,
22296,
74,
48774,
58,
16,
60,
628,
220,
220,
220,
2488,
9288,
2617,
366,
38,
5777,
32096,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
1280,
7,
38,
5777,
13,
34379,
11,
366,
20688,
13,
70,
487,
4943,
466,
266,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3551,
7,
86,
11,
442,
81,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
308,
487,
796,
2824,
7,
9654,
7,
38,
5777,
13,
33634,
11,
366,
20688,
13,
70,
487,
48774,
58,
16,
60,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
308,
65,
74,
29325,
796,
314,
9864,
13712,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
308,
487,
29325,
796,
314,
9864,
13712,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3601,
7,
22296,
74,
29325,
11,
442,
81,
13,
5235,
274,
58,
17,
25,
19,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3601,
7,
70,
487,
29325,
11,
308,
487,
13,
5235,
274,
58,
17,
25,
19,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
10903,
7,
20657,
0,
7,
22296,
74,
29325,
4008,
6624,
10903,
7,
20657,
0,
7,
70,
487,
29325,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
11627,
1631,
5050,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
4129,
7,
354,
81,
13,
5235,
274,
58,
16,
12962,
6624,
4129,
7,
43167,
7,
354,
81,
13,
5235,
274,
58,
16,
60,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
4129,
7,
354,
81,
13,
43167,
8,
6624,
4129,
7,
43167,
7,
354,
81,
4008,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
39358,
6608,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
4129,
7,
26745,
14933,
7,
354,
81,
13,
5235,
274,
58,
16,
60,
4008,
6624,
1105,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
442,
81,
13,
5235,
274,
58,
17,
4083,
75,
10901,
62,
12985,
6624,
366,
12985,
486,
1,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
357,
354,
81,
13,
5235,
274,
58,
17,
4083,
75,
10901,
62,
12985,
796,
366,
12985,
486,
4943,
6624,
366,
12985,
486,
1,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
442,
81,
13,
5235,
274,
58,
17,
4083,
9288,
796,
366,
3791,
5721,
1,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
442,
81,
13,
5235,
274,
58,
17,
4083,
9288,
6624,
366,
3791,
5721,
1,
11405,
477,
7,
1042,
747,
278,
11,
442,
81,
13,
5235,
274,
30109,
16,
11,
18,
11,
19,
11,
20,
11,
21,
60,
4083,
9288,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
651,
7,
354,
81,
13,
5235,
274,
58,
16,
4357,
1058,
75,
10901,
62,
12985,
11,
366,
4943,
6624,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
651,
7,
354,
81,
13,
5235,
274,
58,
17,
4357,
1058,
75,
10901,
62,
12985,
11,
366,
4943,
6624,
366,
12985,
486,
1,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
651,
7,
354,
81,
13,
5235,
274,
58,
17,
4357,
1058,
17946,
436,
363,
11,
366,
4943,
6624,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
5215,
10179,
2025,
30078,
13,
14689,
26745,
0,
7,
354,
81,
13,
5235,
274,
58,
17,
4357,
1058,
9945,
62,
87,
5420,
11,
366,
18878,
25,
10163,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
442,
81,
13,
5235,
274,
58,
17,
4083,
9945,
62,
87,
5420,
6624,
14631,
18878,
25,
18741,
2623,
1415,
1600,
366,
18878,
25,
10163,
8973,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
5215,
10179,
2025,
30078,
13,
31364,
786,
7,
38176,
90,
43730,
11,
5317,
92,
58,
16,
11,
16,
11,
16,
12962,
6624,
16410,
16,
38430,
16,
38430,
16,
11907,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
29993,
341,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
4129,
26933,
70,
13,
75,
10901,
62,
12985,
329,
308,
287,
442,
81,
13,
5235,
274,
12962,
6624,
767,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
32901,
14,
2787,
5165,
10812,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
751,
70,
1734,
0,
7,
354,
81,
11,
1058,
34,
5258,
11,
406,
10901,
7,
6200,
25,
25964,
11,
705,
10,
33809,
1179,
385,
62,
12985,
796,
366,
12985,
3023,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
442,
81,
13,
5235,
274,
58,
437,
4083,
75,
10901,
62,
12985,
6624,
366,
12985,
3023,
1,
198,
220,
220,
220,
220,
220,
220,
220,
12233,
0,
7,
354,
81,
13,
5235,
274,
58,
437,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
442,
81,
13,
5235,
274,
58,
437,
4083,
75,
10901,
62,
12985,
6624,
366,
2301,
486,
1,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
44212,
5235,
274,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
4129,
7,
24592,
7,
31,
5235,
274,
7,
354,
81,
11,
327,
5258,
828,
2488,
5235,
274,
7,
354,
81,
11,
5145,
34,
5258,
22305,
6624,
4129,
7,
354,
81,
13,
5235,
274,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
4129,
7,
3849,
8831,
7,
31,
5235,
274,
7,
354,
81,
11,
327,
5258,
828,
2488,
5235,
274,
7,
354,
81,
11,
5145,
34,
5258,
22305,
6624,
657,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
4129,
7,
24592,
7,
31,
5235,
274,
7,
354,
81,
11,
9779,
828,
2488,
5235,
274,
7,
354,
81,
11,
5145,
70,
1734,
22305,
6624,
4129,
7,
354,
81,
13,
5235,
274,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
4129,
7,
3849,
8831,
7,
31,
5235,
274,
7,
354,
81,
11,
9779,
828,
2488,
5235,
274,
7,
354,
81,
11,
5145,
70,
1734,
22305,
6624,
657,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
4129,
7,
31,
5235,
274,
7,
354,
81,
4008,
6624,
4129,
7,
354,
81,
13,
5235,
274,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
5235,
274,
7,
354,
81,
11,
3895,
7,
70,
1734,
8,
6624,
720,
25,
34,
5258,
8,
6624,
442,
81,
13,
5235,
274,
30109,
17,
11,
19,
11,
21,
11907,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
5235,
274,
7,
354,
81,
11,
3895,
7,
70,
1734,
8,
6624,
720,
25,
34,
5258,
8,
6624,
2488,
5235,
274,
7,
354,
81,
11,
327,
5258,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
5235,
274,
7,
354,
81,
11,
318,
785,
26908,
7,
70,
1734,
4008,
6624,
442,
81,
13,
5235,
274,
30109,
20,
11,
21,
11,
22,
11907,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
5235,
274,
7,
354,
81,
11,
3895,
7,
70,
1734,
8,
6624,
720,
25,
34,
5258,
11,
5145,
271,
785,
26908,
7,
70,
1734,
4008,
6624,
442,
81,
13,
5235,
274,
30109,
17,
11,
19,
11907,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
5235,
274,
7,
354,
81,
11,
4129,
7,
70,
1734,
8,
1279,
5867,
38381,
16,
60,
6624,
442,
81,
13,
5235,
274,
58,
17,
60,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
4129,
7,
31,
5235,
274,
7,
354,
81,
11,
651,
7,
70,
1734,
11,
1058,
75,
10901,
62,
12985,
11,
366,
4943,
6624,
13538,
4008,
6624,
513,
198,
220,
220,
220,
220,
220,
220,
220,
9779,
796,
442,
81,
13,
5235,
274,
58,
18,
60,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
5235,
274,
7,
354,
81,
11,
9779,
6624,
720,
70,
1734,
38381,
16,
60,
6624,
442,
81,
13,
5235,
274,
58,
18,
60,
198,
220,
220,
220,
220,
220,
220,
220,
288,
796,
360,
713,
7,
25,
64,
5218,
366,
12985,
486,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
5235,
274,
7,
354,
81,
11,
1058,
75,
10901,
62,
12985,
6624,
288,
58,
3,
25,
64,
12962,
6624,
2488,
5235,
274,
7,
354,
81,
11,
1058,
75,
10901,
62,
12985,
6624,
366,
12985,
486,
4943,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
30507,
2701,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
44244,
276,
16237,
319,
4683,
3119,
198,
220,
220,
220,
220,
220,
220,
220,
442,
81,
13,
5235,
274,
58,
18,
25,
19,
4083,
70,
1734,
764,
28,
366,
25922,
43,
17,
47,
1,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
477,
7,
354,
81,
13,
5235,
274,
58,
18,
25,
19,
4083,
70,
1734,
764,
855,
366,
25922,
43,
17,
47,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
44244,
276,
16237,
319,
4271,
4814,
3119,
198,
220,
220,
220,
220,
220,
220,
220,
442,
81,
13,
5235,
274,
58,
18,
25,
19,
4083,
3605,
26745,
764,
28,
2081,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
477,
7,
354,
81,
13,
5235,
274,
58,
18,
25,
19,
4083,
3605,
26745,
764,
855,
2081,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
44244,
276,
16237,
351,
2488,
5235,
274,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
5235,
274,
7,
354,
81,
11,
9779,
737,
3605,
26745,
764,
28,
3991,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
477,
7,
354,
81,
13,
5235,
274,
30109,
18,
11,
20,
60,
4083,
3605,
26745,
764,
855,
3991,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
43,
10901,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
1179,
796,
406,
10901,
7,
16,
25,
16,
11,
705,
2637,
11,
2081,
11,
2081,
11,
11801,
17257,
90,
5317,
92,
58,
4357,
3991,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
406,
10901,
3419,
6624,
1179,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
1179,
385,
7,
354,
81,
13,
5235,
274,
58,
17,
12962,
1279,
1179,
385,
7,
354,
81,
13,
5235,
274,
58,
19,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
1179,
385,
7,
354,
81,
13,
5235,
274,
58,
17,
12962,
6624,
1179,
385,
7,
354,
81,
13,
5235,
274,
58,
17,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
318,
785,
26908,
7,
354,
81,
13,
5235,
274,
58,
17,
12962,
6624,
3991,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
318,
785,
26908,
7,
354,
81,
13,
5235,
274,
58,
20,
12962,
6624,
2081,
198,
220,
220,
220,
886,
628,
220,
220,
220,
33756,
796,
288,
2616,
1,
265,
13655,
535,
265,
265,
22260,
330,
1130,
83,
265,
310,
535,
330,
310,
66,
9460,
926,
12985,
265,
310,
6888,
22260,
330,
1130,
64,
4134,
1078,
70,
535,
70,
330,
265,
70,
363,
330,
363,
926,
9460,
83,
265,
66,
13655,
66,
70,
363,
363,
926,
22260,
363,
310,
46071,
330,
70,
363,
66,
363,
12985,
23047,
363,
310,
310,
70,
9246,
310,
4908,
363,
535,
70,
310,
4908,
363,
926,
310,
529,
64,
363,
1130,
83,
1130,
1045,
330,
265,
9246,
535,
13655,
70,
6888,
363,
43552,
8126,
4134,
70,
13227,
265,
363,
22260,
330,
265,
265,
13655,
7252,
1,
198,
220,
220,
220,
2488,
9288,
8379,
7,
354,
81,
13,
5235,
274,
58,
17,
12962,
6624,
33756,
198,
220,
220,
220,
2488,
9288,
4129,
7,
354,
81,
13,
5235,
274,
58,
17,
12962,
6624,
4129,
7,
41068,
8,
628,
220,
220,
220,
2488,
9288,
2617,
366,
40613,
13266,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
442,
81,
796,
5215,
28650,
13,
23739,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
442,
81,
13,
3672,
6624,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
442,
81,
13,
43167,
6624,
288,
2616,
15931,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
442,
81,
13,
25677,
6624,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
442,
81,
13,
5235,
274,
6624,
13005,
21737,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
3891,
7,
354,
81,
13,
70,
2945,
1045,
8,
6624,
17635,
198,
220,
220,
220,
886,
198,
437,
198
] | 1.959629 | 2,477 |
"""
CHELSA{BioClim} <: RasterDataSource
Data from CHELSA, currently only the `BioClim` layer is implemented.
See: [chelsa-climate.org](https://chelsa-climate.org/)
"""
struct CHELSA{X} <: RasterDataSource end
layers(::Type{CHELSA{BioClim}}) = 1:19
"""
getraster(T::Type{CHELSA{BioClim}}, [layer::Integer]) => String
Download CHELSA BioClim data, choosing layers from: `$(layers(CHELSA{BioClim}))`.
Without a layer argument, all layers will be downloaded, and a tuple of paths is returned.
If the data is already downloaded the path will be returned.
"""
function getraster(T::Type{CHELSA{BioClim}}, layer::Integer)
_check_layer(T, layer)
path = rasterpath(T, layer)
url = rasterurl(T, layer)
return _maybe_download(url, path)
end
rastername(::Type{CHELSA{BioClim}}, layer::Integer) = "CHELSA_bio10_$(lpad(layer, 2, "0")).tif"
rasterpath(::Type{CHELSA}) = joinpath(rasterpath(), "CHELSA")
rasterpath(::Type{CHELSA{BioClim}}) = joinpath(rasterpath(CHELSA), "BioClim")
rasterpath(T::Type{CHELSA{BioClim}}, layer::Integer) = joinpath(rasterpath(T), rastername(T, layer))
# https://os.zhdk.cloud.switch.ch/envicloud/chelsa/chelsa_V1/climatologies/bio/CHELSA_prec_01_V1.2_land.tif
rasterurl(::Type{CHELSA}) = URI(scheme="https", host="os.zhdk.cloud.switch.ch", path="/envicloud/chelsa/chelsa_V1/")
rasterurl(::Type{CHELSA{BioClim}}) = joinpath(rasterurl(CHELSA), "climatologies/bio/")
rasterurl(T::Type{CHELSA{BioClim}}, layer::Integer) = joinpath(rasterurl(T), rastername(T, layer))
| [
37811,
198,
220,
220,
220,
5870,
3698,
4090,
90,
42787,
34,
2475,
92,
1279,
25,
371,
1603,
6601,
7416,
198,
198,
6601,
422,
5870,
3698,
4090,
11,
3058,
691,
262,
4600,
42787,
34,
2475,
63,
7679,
318,
9177,
13,
198,
198,
6214,
25,
685,
2395,
7278,
64,
12,
42570,
13,
2398,
16151,
5450,
1378,
2395,
7278,
64,
12,
42570,
13,
2398,
34729,
198,
37811,
198,
7249,
5870,
3698,
4090,
90,
55,
92,
1279,
25,
371,
1603,
6601,
7416,
886,
198,
198,
75,
6962,
7,
3712,
6030,
90,
3398,
3698,
4090,
90,
42787,
34,
2475,
11709,
8,
796,
352,
25,
1129,
198,
198,
37811,
198,
220,
220,
220,
651,
81,
1603,
7,
51,
3712,
6030,
90,
3398,
3698,
4090,
90,
42787,
34,
2475,
92,
5512,
685,
29289,
3712,
46541,
12962,
5218,
10903,
198,
198,
10002,
5870,
3698,
4090,
16024,
34,
2475,
1366,
11,
11236,
11685,
422,
25,
4600,
3,
7,
75,
6962,
7,
3398,
3698,
4090,
90,
42787,
34,
2475,
92,
4008,
44646,
198,
198,
16249,
257,
7679,
4578,
11,
477,
11685,
481,
307,
15680,
11,
290,
257,
46545,
286,
13532,
318,
4504,
13,
220,
198,
1532,
262,
1366,
318,
1541,
15680,
262,
3108,
481,
307,
4504,
13,
198,
37811,
198,
8818,
651,
81,
1603,
7,
51,
3712,
6030,
90,
3398,
3698,
4090,
90,
42787,
34,
2475,
92,
5512,
7679,
3712,
46541,
8,
198,
220,
220,
220,
4808,
9122,
62,
29289,
7,
51,
11,
7679,
8,
198,
220,
220,
220,
3108,
796,
374,
1603,
6978,
7,
51,
11,
7679,
8,
198,
220,
220,
220,
19016,
796,
374,
1603,
6371,
7,
51,
11,
7679,
8,
198,
220,
220,
220,
1441,
4808,
25991,
62,
15002,
7,
6371,
11,
3108,
8,
198,
437,
198,
198,
81,
6470,
480,
7,
3712,
6030,
90,
3398,
3698,
4090,
90,
42787,
34,
2475,
92,
5512,
7679,
3712,
46541,
8,
796,
366,
3398,
3698,
4090,
62,
65,
952,
940,
62,
3,
7,
75,
15636,
7,
29289,
11,
362,
11,
366,
15,
4943,
737,
49929,
1,
198,
198,
81,
1603,
6978,
7,
3712,
6030,
90,
3398,
3698,
4090,
30072,
796,
4654,
6978,
7,
81,
1603,
6978,
22784,
366,
3398,
3698,
4090,
4943,
198,
81,
1603,
6978,
7,
3712,
6030,
90,
3398,
3698,
4090,
90,
42787,
34,
2475,
11709,
8,
796,
4654,
6978,
7,
81,
1603,
6978,
7,
3398,
3698,
4090,
828,
366,
42787,
34,
2475,
4943,
198,
81,
1603,
6978,
7,
51,
3712,
6030,
90,
3398,
3698,
4090,
90,
42787,
34,
2475,
92,
5512,
7679,
3712,
46541,
8,
796,
4654,
6978,
7,
81,
1603,
6978,
7,
51,
828,
374,
6470,
480,
7,
51,
11,
7679,
4008,
198,
198,
2,
3740,
1378,
418,
13,
23548,
34388,
13,
17721,
13,
31943,
13,
354,
14,
24330,
291,
75,
2778,
14,
2395,
7278,
64,
14,
2395,
7278,
64,
62,
53,
16,
14,
565,
320,
265,
5823,
14,
65,
952,
14,
3398,
3698,
4090,
62,
3866,
66,
62,
486,
62,
53,
16,
13,
17,
62,
1044,
13,
49929,
198,
81,
1603,
6371,
7,
3712,
6030,
90,
3398,
3698,
4090,
30072,
796,
43975,
7,
15952,
1326,
2625,
5450,
1600,
2583,
2625,
418,
13,
23548,
34388,
13,
17721,
13,
31943,
13,
354,
1600,
3108,
35922,
24330,
291,
75,
2778,
14,
2395,
7278,
64,
14,
2395,
7278,
64,
62,
53,
16,
14,
4943,
198,
81,
1603,
6371,
7,
3712,
6030,
90,
3398,
3698,
4090,
90,
42787,
34,
2475,
11709,
8,
796,
4654,
6978,
7,
81,
1603,
6371,
7,
3398,
3698,
4090,
828,
366,
565,
320,
265,
5823,
14,
65,
952,
14,
4943,
198,
81,
1603,
6371,
7,
51,
3712,
6030,
90,
3398,
3698,
4090,
90,
42787,
34,
2475,
92,
5512,
7679,
3712,
46541,
8,
796,
4654,
6978,
7,
81,
1603,
6371,
7,
51,
828,
374,
6470,
480,
7,
51,
11,
7679,
4008,
198
] | 2.458537 | 615 |
if basename(pwd()) == "aoc"
cd("2018/10")
end
using OffsetArrays
mutable struct LightPoint
position::CartesianIndex{2}
velocity::CartesianIndex{2}
end
struct LightField
points
end
function Base.size(lightfield::LightField)
Tuple(-(reverse(extrema(getfield.(lightfield.points, :position)))...))
end
Base.length(lightfield::LightField) = prod(size(lightfield))
function Base.in(position::CartesianIndex, lightfield::LightField)
any(point.position == position for point in lightfield.points)
end
function loadpoints(filename::AbstractString)
LightField([
begin
a, b, c, d = parse.(Int, m.match for m in eachmatch(r"-?\d+", line))
LightPoint(CartesianIndex(a, b), CartesianIndex(c, d))
end
for line in eachline(filename)
])
end
function step!(lightfield::LightField; rev = false)
foreach(
if rev
point -> point.position -= point.velocity
else
point -> point.position += point.velocity
end,
lightfield.points
)
lightfield
end
function Base.show(io::IO, lightfield::LightField)
println("LightField with ", length(lightfield.points), " points:")
for col in eachcol(range(extrema(getfield.(lightfield.points, :position))...))
println(io, " ", (p in lightfield ? '#' : ' ' for p in col)...)
end
end
part1(filename::AbstractString) = part1(loadpoints(filename))
function part1(lightfield::LightField)
len = length(lightfield)
minlen = len + 1
steps = -1
while minlen > len
minlen = len
len = length(step!(lightfield))
steps += 1
end
step!(lightfield, rev = true), steps
end
| [
361,
1615,
12453,
7,
79,
16993,
28955,
6624,
366,
64,
420,
1,
198,
220,
220,
220,
22927,
7203,
7908,
14,
940,
4943,
198,
437,
198,
3500,
3242,
2617,
3163,
20477,
198,
198,
76,
18187,
2878,
4401,
12727,
198,
220,
220,
220,
2292,
3712,
43476,
35610,
15732,
90,
17,
92,
198,
220,
220,
220,
15432,
3712,
43476,
35610,
15732,
90,
17,
92,
198,
437,
198,
198,
7249,
4401,
15878,
198,
220,
220,
220,
2173,
198,
437,
198,
198,
8818,
7308,
13,
7857,
7,
2971,
3245,
3712,
15047,
15878,
8,
198,
220,
220,
220,
309,
29291,
7,
30420,
50188,
7,
2302,
260,
2611,
7,
1136,
3245,
12195,
2971,
3245,
13,
13033,
11,
1058,
9150,
22305,
986,
4008,
198,
437,
198,
198,
14881,
13,
13664,
7,
2971,
3245,
3712,
15047,
15878,
8,
796,
40426,
7,
7857,
7,
2971,
3245,
4008,
198,
198,
8818,
7308,
13,
259,
7,
9150,
3712,
43476,
35610,
15732,
11,
1657,
3245,
3712,
15047,
15878,
8,
198,
220,
220,
220,
597,
7,
4122,
13,
9150,
6624,
2292,
329,
966,
287,
1657,
3245,
13,
13033,
8,
198,
437,
198,
198,
8818,
3440,
13033,
7,
34345,
3712,
23839,
10100,
8,
198,
220,
220,
220,
4401,
15878,
26933,
198,
220,
220,
220,
220,
220,
220,
220,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
257,
11,
275,
11,
269,
11,
288,
796,
21136,
12195,
5317,
11,
285,
13,
15699,
329,
285,
287,
1123,
15699,
7,
81,
26793,
30,
59,
67,
10,
1600,
1627,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4401,
12727,
7,
43476,
35610,
15732,
7,
64,
11,
275,
828,
13690,
35610,
15732,
7,
66,
11,
288,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
329,
1627,
287,
1123,
1370,
7,
34345,
8,
198,
220,
220,
220,
33761,
198,
437,
198,
198,
8818,
2239,
0,
7,
2971,
3245,
3712,
15047,
15878,
26,
2710,
796,
3991,
8,
198,
220,
220,
220,
1674,
620,
7,
198,
220,
220,
220,
220,
220,
220,
220,
611,
2710,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
966,
4613,
966,
13,
9150,
48185,
966,
13,
626,
11683,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
966,
4613,
966,
13,
9150,
15853,
966,
13,
626,
11683,
198,
220,
220,
220,
220,
220,
220,
220,
886,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1657,
3245,
13,
13033,
198,
220,
220,
220,
1267,
198,
220,
220,
220,
1657,
3245,
198,
437,
198,
198,
8818,
7308,
13,
12860,
7,
952,
3712,
9399,
11,
1657,
3245,
3712,
15047,
15878,
8,
198,
220,
220,
220,
44872,
7203,
15047,
15878,
351,
33172,
4129,
7,
2971,
3245,
13,
13033,
828,
366,
2173,
25,
4943,
198,
220,
220,
220,
329,
951,
287,
1123,
4033,
7,
9521,
7,
2302,
260,
2611,
7,
1136,
3245,
12195,
2971,
3245,
13,
13033,
11,
1058,
9150,
4008,
986,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
44872,
7,
952,
11,
366,
220,
33172,
357,
79,
287,
1657,
3245,
5633,
705,
2,
6,
1058,
705,
705,
329,
279,
287,
951,
8,
23029,
198,
220,
220,
220,
886,
198,
437,
198,
198,
3911,
16,
7,
34345,
3712,
23839,
10100,
8,
796,
636,
16,
7,
2220,
13033,
7,
34345,
4008,
198,
8818,
636,
16,
7,
2971,
3245,
3712,
15047,
15878,
8,
198,
220,
220,
220,
18896,
796,
4129,
7,
2971,
3245,
8,
198,
220,
220,
220,
949,
11925,
796,
18896,
1343,
352,
198,
220,
220,
220,
4831,
796,
532,
16,
198,
220,
220,
220,
981,
949,
11925,
1875,
18896,
198,
220,
220,
220,
220,
220,
220,
220,
949,
11925,
796,
18896,
198,
220,
220,
220,
220,
220,
220,
220,
18896,
796,
4129,
7,
9662,
0,
7,
2971,
3245,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
4831,
15853,
352,
198,
220,
220,
220,
886,
198,
220,
220,
220,
2239,
0,
7,
2971,
3245,
11,
2710,
796,
2081,
828,
4831,
198,
437,
198
] | 2.526158 | 669 |
using memoJuliaClassJa
using Documenter
## Adopted from docs/make.jl in Documenter.jl
# The DOCSARGS environment variable can be used to pass additional arguments to make.jl.
# This is useful on CI, if you need to change the behavior of the build slightly but you
# can not change the .travis.yml or make.jl scripts any more (e.g. for a tag build).
if haskey(ENV, "DOCSARGS")
for arg in split(ENV["DOCSARGS"])
(arg in ARGS) || push!(ARGS, arg)
end
end
makedocs(
modules=[memoJuliaClassJa],
authors="Hiroharu Sugawara <hsugawa@gmail.com>",
clean = false,
sitename="memoJuliaClassJa.jl",
format=Documenter.HTML(;
prettyurls=get(ENV, "CI", "false") == "true",
canonical="https://hsugawa8651.github.io/memoJuliaClassJa.jl/stable/",
assets=String[],
),
linkcheck = !("skiplinks" in ARGS),
linkcheck_ignore = [
# timelessrepo.com seems to 404 on any CURL request...
"http://timelessrepo.com/json-isnt-a-javascript-subset",
# We'll ignore links that point to GitHub's edit pages, as they redirect to the
# login screen and cause a warning:
r"https://github.com/([A-Za-z0-9_.-]+)/([A-Za-z0-9_.-]+)/edit(.*)",
] ∪ (get(ENV, "GITHUB_ACTIONS", nothing) == "true" ? [
# Extra ones we ignore only on CI.
#
# It seems that CTAN blocks GitHub Actions?
"https://ctan.org/pkg/minted",
] : []),
pages= [
"Home" => "index.md",
"LICENSE.md",
"LICENSEja.md",
"ch00.md",
"ch01.md",
"ch02.md",
"ch03.md",
"ch04.md",
"ch05.md",
"ch06.md",
"ch07.md",
"ch08.md",
"ch09.md",
"ch10.md",
"ch11.md",
"ch12.md",
"ch13.md",
"porting.md"
],
# strict = !("strict=false" in ARGS),
doctest = ("doctest=only" in ARGS) ? :only : true,
)
deploydocs(
repo="github.com/hsugawa8651/memoJuliaClassJa.jl.git",
devbranch="develop",
push_preview = true,
)
| [
3500,
16155,
16980,
544,
9487,
33186,
198,
3500,
16854,
263,
198,
198,
2235,
1215,
45256,
422,
34165,
14,
15883,
13,
20362,
287,
16854,
263,
13,
20362,
198,
2,
383,
37760,
50,
1503,
14313,
2858,
7885,
460,
307,
973,
284,
1208,
3224,
7159,
284,
787,
13,
20362,
13,
198,
2,
770,
318,
4465,
319,
14514,
11,
611,
345,
761,
284,
1487,
262,
4069,
286,
262,
1382,
4622,
475,
345,
198,
2,
460,
407,
1487,
262,
764,
83,
16956,
13,
88,
4029,
393,
787,
13,
20362,
14750,
597,
517,
357,
68,
13,
70,
13,
329,
257,
7621,
1382,
737,
198,
361,
468,
2539,
7,
1677,
53,
11,
366,
38715,
50,
1503,
14313,
4943,
198,
220,
220,
220,
329,
1822,
287,
6626,
7,
1677,
53,
14692,
38715,
50,
1503,
14313,
8973,
8,
198,
220,
220,
220,
220,
220,
220,
220,
357,
853,
287,
5923,
14313,
8,
8614,
4574,
0,
7,
1503,
14313,
11,
1822,
8,
198,
220,
220,
220,
886,
198,
437,
198,
198,
76,
4335,
420,
82,
7,
198,
220,
220,
220,
13103,
41888,
11883,
78,
16980,
544,
9487,
33186,
4357,
198,
220,
220,
220,
7035,
2625,
39,
7058,
9869,
84,
13691,
707,
3301,
1279,
11994,
1018,
6909,
31,
14816,
13,
785,
29,
1600,
198,
220,
220,
220,
3424,
796,
3991,
11,
198,
220,
220,
220,
1650,
12453,
2625,
11883,
78,
16980,
544,
9487,
33186,
13,
20362,
1600,
198,
220,
220,
220,
5794,
28,
24941,
263,
13,
28656,
7,
26,
198,
220,
220,
220,
220,
220,
220,
220,
2495,
6371,
82,
28,
1136,
7,
1677,
53,
11,
366,
25690,
1600,
366,
9562,
4943,
6624,
366,
7942,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
40091,
2625,
5450,
1378,
11994,
1018,
6909,
23,
40639,
13,
12567,
13,
952,
14,
11883,
78,
16980,
544,
9487,
33186,
13,
20362,
14,
31284,
14,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
6798,
28,
10100,
58,
4357,
198,
220,
220,
220,
10612,
198,
220,
220,
220,
2792,
9122,
796,
5145,
7203,
20545,
489,
2973,
1,
287,
5923,
14313,
828,
198,
220,
220,
220,
2792,
9122,
62,
46430,
796,
685,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
36464,
260,
7501,
13,
785,
2331,
284,
32320,
319,
597,
327,
21886,
2581,
986,
198,
220,
220,
220,
220,
220,
220,
220,
366,
4023,
1378,
16514,
5321,
260,
7501,
13,
785,
14,
17752,
12,
271,
429,
12,
64,
12,
37495,
12,
7266,
2617,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
775,
1183,
8856,
6117,
326,
966,
284,
21722,
338,
4370,
5468,
11,
355,
484,
18941,
284,
262,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
17594,
3159,
290,
2728,
257,
6509,
25,
198,
220,
220,
220,
220,
220,
220,
220,
374,
1,
5450,
1378,
12567,
13,
785,
14,
26933,
32,
12,
57,
64,
12,
89,
15,
12,
24,
62,
7874,
48688,
20679,
26933,
32,
12,
57,
64,
12,
89,
15,
12,
24,
62,
7874,
48688,
20679,
19312,
7,
15885,
42501,
198,
220,
220,
220,
2361,
18872,
103,
357,
1136,
7,
1677,
53,
11,
366,
38,
10554,
10526,
62,
10659,
11053,
1600,
2147,
8,
220,
6624,
366,
7942,
1,
5633,
685,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
17221,
3392,
356,
8856,
691,
319,
14514,
13,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
632,
2331,
326,
16356,
1565,
7021,
21722,
24439,
30,
198,
220,
220,
220,
220,
220,
220,
220,
366,
5450,
1378,
310,
272,
13,
2398,
14,
35339,
14,
34289,
276,
1600,
198,
220,
220,
220,
2361,
1058,
17635,
828,
198,
220,
220,
220,
5468,
28,
685,
198,
197,
1,
16060,
1,
5218,
366,
9630,
13,
9132,
1600,
198,
197,
197,
1,
43,
2149,
24290,
13,
9132,
1600,
198,
197,
197,
1,
43,
2149,
24290,
6592,
13,
9132,
1600,
198,
197,
197,
1,
354,
405,
13,
9132,
1600,
198,
197,
197,
1,
354,
486,
13,
9132,
1600,
198,
197,
197,
1,
354,
2999,
13,
9132,
1600,
198,
197,
197,
1,
354,
3070,
13,
9132,
1600,
198,
197,
197,
1,
354,
3023,
13,
9132,
1600,
198,
197,
197,
1,
354,
2713,
13,
9132,
1600,
198,
197,
197,
1,
354,
3312,
13,
9132,
1600,
198,
197,
197,
1,
354,
2998,
13,
9132,
1600,
198,
197,
197,
1,
354,
2919,
13,
9132,
1600,
198,
197,
197,
1,
354,
2931,
13,
9132,
1600,
198,
197,
197,
1,
354,
940,
13,
9132,
1600,
198,
197,
197,
1,
354,
1157,
13,
9132,
1600,
198,
197,
197,
1,
354,
1065,
13,
9132,
1600,
198,
197,
197,
1,
354,
1485,
13,
9132,
1600,
198,
197,
197,
1,
26527,
13,
9132,
1,
198,
197,
4357,
198,
220,
220,
1303,
7646,
796,
5145,
7203,
301,
2012,
28,
9562,
1,
287,
5923,
14313,
828,
198,
220,
220,
10412,
395,
796,
5855,
4598,
310,
395,
28,
8807,
1,
287,
5923,
14313,
8,
5633,
1058,
8807,
1058,
2081,
11,
198,
8,
198,
198,
2934,
1420,
31628,
7,
198,
220,
220,
220,
29924,
2625,
12567,
13,
785,
14,
11994,
1018,
6909,
23,
40639,
14,
11883,
78,
16980,
544,
9487,
33186,
13,
20362,
13,
18300,
1600,
198,
220,
220,
220,
1614,
1671,
3702,
2625,
16244,
1600,
198,
220,
220,
220,
4574,
62,
3866,
1177,
796,
2081,
11,
198,
8,
198
] | 2.224277 | 865 |
function fx(t, x, fx)
fx .= x
end
function fq(t, q, p, fq)
fq .= q
end
function fp(t, q, p, fp)
fp .= q.^2
end
function fϕ(t, x, fϕ)
fϕ .= 0
end
function gx(t, x, λ, fx)
fx .= x
end
function gq(t, q, p, λ, fλ)
fλ .= q
end
function gp(t, q, p, λ, fλ)
fλ .= q.^2
end
function gϕ(t, q, p, gϕ)
gϕ .= p - q.^2
end
| [
198,
8818,
277,
87,
7,
83,
11,
2124,
11,
277,
87,
8,
198,
220,
220,
220,
277,
87,
764,
28,
2124,
198,
437,
198,
198,
8818,
277,
80,
7,
83,
11,
10662,
11,
279,
11,
277,
80,
8,
198,
220,
220,
220,
277,
80,
764,
28,
10662,
198,
437,
198,
198,
8818,
277,
79,
7,
83,
11,
10662,
11,
279,
11,
277,
79,
8,
198,
220,
220,
220,
277,
79,
764,
28,
10662,
13,
61,
17,
198,
437,
198,
198,
8818,
277,
139,
243,
7,
83,
11,
2124,
11,
277,
139,
243,
8,
198,
220,
220,
220,
277,
139,
243,
764,
28,
657,
198,
437,
198,
198,
8818,
308,
87,
7,
83,
11,
2124,
11,
7377,
119,
11,
277,
87,
8,
198,
220,
220,
220,
277,
87,
764,
28,
2124,
198,
437,
198,
198,
8818,
308,
80,
7,
83,
11,
10662,
11,
279,
11,
7377,
119,
11,
277,
39377,
8,
198,
220,
220,
220,
277,
39377,
764,
28,
10662,
198,
437,
198,
198,
8818,
27809,
7,
83,
11,
10662,
11,
279,
11,
7377,
119,
11,
277,
39377,
8,
198,
220,
220,
220,
277,
39377,
764,
28,
10662,
13,
61,
17,
198,
437,
198,
198,
8818,
308,
139,
243,
7,
83,
11,
10662,
11,
279,
11,
308,
139,
243,
8,
198,
220,
220,
220,
308,
139,
243,
764,
28,
279,
532,
10662,
13,
61,
17,
198,
437,
198
] | 1.537445 | 227 |
using CompilerOptions
using Base.Test
JLOptions()
| [
3500,
3082,
5329,
29046,
198,
3500,
7308,
13,
14402,
198,
198,
41,
21982,
8544,
3419,
198
] | 3.1875 | 16 |
# using DelimitedFiles
# const UCR_DATASETS = """
# 50words,
# Adiac,
# ArrowHead,
# Beef,
# BeetleFly,
# BirdChicken,
# CBF,
# Car,
# ChlorineConcentration,
# CinC_ECG_torso,
# Coffee,
# Computers,
# Cricket_X,
# Cricket_Y,
# Cricket_Z,
# DiatomSizeReduction,
# DistalPhalanxOutlineAgeGroup,
# DistalPhalanxOutlineCorrect,
# DistalPhalanxTW,
# ECG200,
# ECG5000,
# ECGFiveDays,
# Earthquakes,
# ElectricDevices,
# FISH,
# FaceAll,
# FaceFour,
# FacesUCR,
# FordA,
# FordB,
# Gun_Point,
# Ham,
# HandOutlines,
# Haptics,
# Herring,
# InlineSkate,
# InsectWingbeatSound,
# ItalyPowerDemand,
# LargeKitchenAppliances,
# Lighting2,
# Lighting7,
# MALLAT,
# Meat,
# MedicalImages,
# MiddlePhalanxOutlineAgeGroup,
# MiddlePhalanxOutlineCorrect,
# MiddlePhalanxTW,
# MoteStrain,
# NonInvasiveFatalECG_Thorax1,
# NonInvasiveFatalECG_Thorax2,
# OSULeaf,
# OliveOil,
# PhalangesOutlinesCorrect,
# Phoneme,
# Plane,
# ProximalPhalanxOutlineAgeGroup,
# ProximalPhalanxOutlineCorrect,
# ProximalPhalanxTW,
# RefrigerationDevices,
# ScreenType,
# ShapeletSim,
# ShapesAll,
# SmallKitchenAppliances,
# SonyAIBORobotSurface,
# SonyAIBORobotSurfaceII,
# StarLightCurves,
# Strawberry,
# SwedishLeaf,
# Symbols,
# ToeSegmentation1,
# ToeSegmentation2,
# Trace,
# TwoLeadECG,
# Two_Patterns,
# UWaveGestureLibraryAll,
# Wine,
# WordsSynonyms,
# Worms,
# WormsTwoClass,
# synthetic_control,
# uWaveGestureLibrary_X,
# uWaveGestureLibrary_Y,
# uWaveGestureLibrary_Z,
# wafer,
# yoga
# """
#
# """
# DynamicAxisWarping.download_data([path="/DynamicAxisWarping/data/"])
#
# Downloads the UC Riverside Time Series Classification Archive to
# the specified path.
# """
# function download_ucr()
#
# UCR_URL = "http://www.cs.ucr.edu/~eamonn/time_series_data/UCR_TS_Archive_2015.zip"
#
# # the password is "attempttoclassify"
# @info(
# """
# A simple password is required to extract the UCR Time Series Classification. While the
# data is downloading, please read the following paper
#
# Bing Hu, Yanping Chen, Eamonn J. Keogh: Time Series Classification under More Realistic
# Assumptions. SDM 2013: 578-586.
# http://dx.doi.org/10.1137/1.9781611972832.64
#
# Find the following sentence (it's near the end of the first page):
#
# “Every item that we ******* ## @@@@@@@ belongs to exactly one of our well defined classes”
#
# Enter the three redacted words (without spaces) to extract the data. The purpose of this
# exercise is to encourage you to read the associated paper, see:
#
# http://www.cs.ucr.edu/~eamonn/time_series_data/
#
# """
# )
#
# download(UCR_URL, "$DATAPATH/UCR.zip")
#
# # should unzip the file correctly in platform-specific manner
# run(unpack_cmd("UCR", DATAPATH , ".zip", ""))
#
# # delete the zip file when we're done
# rm("$DATAPATH/UCR.zip")
#
# @info("Download and extraction successful!")
#
# end
#
# """
# data,labels = DynamicAxisWarping.traindata(name)
#
# Loads the training set of the specified dataset. Returns a matrix `data` where each column
# holds a 1-dimensional time series. The class label for each column is held `labels`
# which is a vector of length `size(data,2)`.
#
# Available datasets:
#
# $UCR_DATASETS
# """
# function ucr_traindata(name::AbstractString)
# try
# Y = readdlm(DATAPATH*"/UCR_TS_Archive_2015/"*name*"/"*name*"_TRAIN", ',')
# labels = round(Int,vec(Y[:,1]))
# data = transpose(Y[:,2:end])
# return data,labels
# catch err
# showerror(stdout, err, backtrace());println()
# @info("You may have recieved this error because you haven't downloaded the database yet.")
# @info("Try running download_ucr() first.")
# @info("You may also have mispelled the name of the dataset.")
# end
# end
#
# """
# data,labels = DynamicAxisWarping.traindata(name)
#
# Loads the test set of the specified dataset. Returns a matrix `data` where each column
# holds a 1-dimensional time series. The class label for each column is held `labels`
# which is a vector of length `size(data,2)`.
#
# Available datasets:
#
# $UCR_DATASETS
# """
# function ucr_testdata(name::AbstractString)
# try
# Y = return readcsv(DATAPATH*"/UCR_TS_Archive_2015/"*name*"/"*name*"_TEST")
# labels = round(Int,vec(Y[:,1]))
# data = transpose(Y[:,2:end])
# return data,labels
# catch err
# showerror(stdout, err, backtrace());println()
# @info("You may have recieved this error because you haven't downloaded the database yet.")
# @info("Try running download_ucr() first.")
# @info("You may also have mispelled the name of the dataset.")
# end
# end
| [
2,
1262,
4216,
320,
863,
25876,
198,
2,
1500,
471,
9419,
62,
35,
1404,
1921,
32716,
796,
37227,
198,
2,
2026,
10879,
11,
198,
2,
1215,
9607,
11,
198,
2,
19408,
13847,
11,
198,
2,
35309,
11,
198,
2,
47464,
33771,
11,
198,
2,
14506,
45565,
11,
198,
2,
10078,
37,
11,
198,
2,
1879,
11,
198,
2,
609,
4685,
500,
3103,
1087,
1358,
11,
198,
2,
327,
259,
34,
62,
2943,
38,
62,
13165,
568,
11,
198,
2,
19443,
11,
198,
2,
22476,
364,
11,
198,
2,
34761,
62,
55,
11,
198,
2,
34761,
62,
56,
11,
198,
2,
34761,
62,
57,
11,
198,
2,
360,
5375,
296,
10699,
7738,
8110,
11,
198,
2,
4307,
282,
2725,
25786,
87,
7975,
1370,
23396,
13247,
11,
198,
2,
4307,
282,
2725,
25786,
87,
7975,
1370,
42779,
11,
198,
2,
4307,
282,
2725,
25786,
87,
34551,
11,
198,
2,
13182,
38,
2167,
11,
198,
2,
13182,
38,
27641,
11,
198,
2,
13182,
38,
20029,
38770,
11,
198,
2,
33842,
1124,
11,
198,
2,
13944,
13603,
1063,
11,
198,
2,
376,
18422,
11,
198,
2,
15399,
3237,
11,
198,
2,
15399,
15137,
11,
198,
2,
48463,
52,
9419,
11,
198,
2,
8092,
32,
11,
198,
2,
8092,
33,
11,
198,
2,
6748,
62,
12727,
11,
198,
2,
4345,
11,
198,
2,
7157,
7975,
6615,
11,
198,
2,
367,
2373,
873,
11,
198,
2,
2332,
1806,
11,
198,
2,
554,
1370,
15739,
378,
11,
198,
2,
36652,
35612,
12945,
21369,
11,
198,
2,
8031,
13434,
42782,
11,
198,
2,
13601,
20827,
6607,
4677,
75,
16097,
11,
198,
2,
43150,
17,
11,
198,
2,
43150,
22,
11,
198,
2,
337,
7036,
1404,
11,
198,
2,
19145,
11,
198,
2,
8366,
29398,
11,
198,
2,
6046,
2725,
25786,
87,
7975,
1370,
23396,
13247,
11,
198,
2,
6046,
2725,
25786,
87,
7975,
1370,
42779,
11,
198,
2,
6046,
2725,
25786,
87,
34551,
11,
198,
2,
337,
1258,
1273,
3201,
11,
198,
2,
8504,
818,
23747,
37,
10254,
2943,
38,
62,
46765,
897,
16,
11,
198,
2,
8504,
818,
23747,
37,
10254,
2943,
38,
62,
46765,
897,
17,
11,
198,
2,
7294,
52,
3123,
1878,
11,
198,
2,
30012,
44142,
11,
198,
2,
1380,
282,
6231,
7975,
6615,
42779,
11,
198,
2,
1380,
261,
34755,
11,
198,
2,
36829,
11,
198,
2,
1041,
87,
4402,
2725,
25786,
87,
7975,
1370,
23396,
13247,
11,
198,
2,
1041,
87,
4402,
2725,
25786,
87,
7975,
1370,
42779,
11,
198,
2,
1041,
87,
4402,
2725,
25786,
87,
34551,
11,
198,
2,
6524,
18096,
341,
13603,
1063,
11,
198,
2,
15216,
6030,
11,
198,
2,
25959,
1616,
8890,
11,
198,
2,
911,
7916,
3237,
11,
198,
2,
10452,
20827,
6607,
4677,
75,
16097,
11,
198,
2,
10184,
32,
9865,
1581,
672,
313,
14214,
2550,
11,
198,
2,
10184,
32,
9865,
1581,
672,
313,
14214,
2550,
3978,
11,
198,
2,
2907,
15047,
26628,
1158,
11,
198,
2,
42611,
11,
198,
2,
14023,
3123,
1878,
11,
198,
2,
41327,
10220,
11,
198,
2,
1675,
68,
41030,
14374,
16,
11,
198,
2,
1675,
68,
41030,
14374,
17,
11,
198,
2,
34912,
11,
198,
2,
4930,
20451,
2943,
38,
11,
198,
2,
4930,
62,
47546,
82,
11,
198,
2,
33436,
1015,
38,
395,
495,
23377,
3237,
11,
198,
2,
20447,
11,
198,
2,
23087,
29934,
43612,
11,
198,
2,
28505,
82,
11,
198,
2,
28505,
82,
7571,
9487,
11,
198,
2,
18512,
62,
13716,
11,
198,
2,
334,
39709,
38,
395,
495,
23377,
62,
55,
11,
198,
2,
334,
39709,
38,
395,
495,
23377,
62,
56,
11,
198,
2,
334,
39709,
38,
395,
495,
23377,
62,
57,
11,
198,
2,
266,
34659,
11,
198,
2,
20351,
198,
2,
37227,
198,
2,
198,
2,
37227,
198,
2,
220,
220,
220,
220,
26977,
31554,
271,
54,
5117,
278,
13,
15002,
62,
7890,
26933,
6978,
35922,
44090,
31554,
271,
54,
5117,
278,
14,
7890,
14,
8973,
8,
198,
2,
198,
2,
50093,
262,
14417,
35597,
3862,
7171,
40984,
20816,
284,
198,
2,
262,
7368,
3108,
13,
198,
2,
37227,
198,
2,
2163,
4321,
62,
1229,
81,
3419,
198,
2,
198,
2,
220,
220,
220,
220,
471,
9419,
62,
21886,
796,
366,
4023,
1378,
2503,
13,
6359,
13,
1229,
81,
13,
15532,
14,
93,
68,
16487,
77,
14,
2435,
62,
25076,
62,
7890,
14,
52,
9419,
62,
4694,
62,
19895,
425,
62,
4626,
13,
13344,
1,
198,
2,
198,
2,
220,
220,
220,
220,
1303,
262,
9206,
318,
366,
1078,
1791,
40301,
31172,
1958,
1,
198,
2,
220,
220,
220,
220,
2488,
10951,
7,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
37227,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
317,
2829,
9206,
318,
2672,
284,
7925,
262,
471,
9419,
3862,
7171,
40984,
13,
2893,
262,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
1366,
318,
22023,
11,
3387,
1100,
262,
1708,
3348,
198,
2,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
21631,
11256,
11,
10642,
13886,
12555,
11,
412,
16487,
77,
449,
13,
3873,
46664,
25,
3862,
7171,
40984,
739,
3125,
6416,
2569,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
2195,
388,
8544,
13,
9834,
44,
2211,
25,
642,
3695,
12,
29796,
13,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
2638,
1378,
34350,
13,
34023,
13,
2398,
14,
940,
13,
1157,
2718,
14,
16,
13,
32196,
1433,
16315,
48524,
2624,
13,
2414,
198,
2,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
9938,
262,
1708,
6827,
357,
270,
338,
1474,
262,
886,
286,
262,
717,
2443,
2599,
198,
2,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
564,
250,
6109,
2378,
326,
356,
25998,
8162,
22492,
2488,
22675,
12404,
14448,
284,
3446,
530,
286,
674,
880,
5447,
6097,
447,
251,
198,
2,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
6062,
262,
1115,
44740,
2456,
357,
19419,
9029,
8,
284,
7925,
262,
1366,
13,
383,
4007,
286,
428,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
5517,
318,
284,
7898,
345,
284,
1100,
262,
3917,
3348,
11,
766,
25,
198,
2,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
2638,
1378,
2503,
13,
6359,
13,
1229,
81,
13,
15532,
14,
93,
68,
16487,
77,
14,
2435,
62,
25076,
62,
7890,
14,
198,
2,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
37227,
198,
2,
220,
220,
220,
220,
1267,
198,
2,
198,
2,
220,
220,
220,
220,
4321,
7,
52,
9419,
62,
21886,
11,
17971,
35,
1404,
2969,
12599,
14,
52,
9419,
13,
13344,
4943,
198,
2,
198,
2,
220,
220,
220,
220,
1303,
815,
555,
13344,
262,
2393,
9380,
287,
3859,
12,
11423,
5642,
198,
2,
220,
220,
220,
220,
1057,
7,
403,
8002,
62,
28758,
7203,
52,
9419,
1600,
360,
1404,
2969,
12599,
837,
27071,
13344,
1600,
13538,
4008,
198,
2,
198,
2,
220,
220,
220,
220,
1303,
12233,
262,
19974,
2393,
618,
356,
821,
1760,
198,
2,
220,
220,
220,
220,
42721,
7203,
3,
35,
1404,
2969,
12599,
14,
52,
9419,
13,
13344,
4943,
198,
2,
198,
2,
220,
220,
220,
220,
2488,
10951,
7203,
10002,
290,
22236,
4388,
2474,
8,
198,
2,
198,
2,
886,
198,
2,
198,
2,
37227,
198,
2,
220,
220,
220,
220,
1366,
11,
23912,
1424,
796,
26977,
31554,
271,
54,
5117,
278,
13,
27432,
7890,
7,
3672,
8,
198,
2,
198,
2,
8778,
82,
262,
3047,
900,
286,
262,
7368,
27039,
13,
16409,
257,
17593,
4600,
7890,
63,
810,
1123,
5721,
198,
2,
6622,
257,
352,
12,
19577,
640,
2168,
13,
383,
1398,
6167,
329,
1123,
5721,
318,
2714,
4600,
23912,
1424,
63,
198,
2,
543,
318,
257,
15879,
286,
4129,
4600,
7857,
7,
7890,
11,
17,
8,
44646,
198,
2,
198,
2,
14898,
40522,
25,
198,
2,
198,
2,
720,
52,
9419,
62,
35,
1404,
1921,
32716,
198,
2,
37227,
198,
2,
2163,
334,
6098,
62,
27432,
7890,
7,
3672,
3712,
23839,
10100,
8,
198,
2,
220,
220,
220,
220,
1949,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
575,
796,
1100,
25404,
76,
7,
35,
1404,
2969,
12599,
9,
1,
14,
52,
9419,
62,
4694,
62,
19895,
425,
62,
4626,
30487,
9,
3672,
9,
1,
30487,
9,
3672,
9,
1,
62,
51,
3861,
1268,
1600,
705,
4032,
8,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
14722,
796,
2835,
7,
5317,
11,
35138,
7,
56,
58,
45299,
16,
60,
4008,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
1366,
796,
1007,
3455,
7,
56,
58,
45299,
17,
25,
437,
12962,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
1441,
1366,
11,
23912,
1424,
198,
2,
220,
220,
220,
220,
4929,
11454,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
14643,
1472,
7,
19282,
448,
11,
11454,
11,
736,
40546,
35430,
35235,
3419,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
10951,
7203,
1639,
743,
423,
664,
39591,
428,
4049,
780,
345,
4398,
470,
15680,
262,
6831,
1865,
19570,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
10951,
7203,
23433,
2491,
4321,
62,
1229,
81,
3419,
717,
19570,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
10951,
7203,
1639,
743,
635,
423,
2984,
15803,
262,
1438,
286,
262,
27039,
19570,
198,
2,
220,
220,
220,
220,
886,
198,
2,
886,
198,
2,
198,
2,
37227,
198,
2,
220,
220,
220,
220,
1366,
11,
23912,
1424,
796,
26977,
31554,
271,
54,
5117,
278,
13,
27432,
7890,
7,
3672,
8,
198,
2,
198,
2,
8778,
82,
262,
1332,
900,
286,
262,
7368,
27039,
13,
16409,
257,
17593,
4600,
7890,
63,
810,
1123,
5721,
198,
2,
6622,
257,
352,
12,
19577,
640,
2168,
13,
383,
1398,
6167,
329,
1123,
5721,
318,
2714,
4600,
23912,
1424,
63,
198,
2,
543,
318,
257,
15879,
286,
4129,
4600,
7857,
7,
7890,
11,
17,
8,
44646,
198,
2,
198,
2,
14898,
40522,
25,
198,
2,
198,
2,
720,
52,
9419,
62,
35,
1404,
1921,
32716,
198,
2,
37227,
198,
2,
2163,
334,
6098,
62,
9288,
7890,
7,
3672,
3712,
23839,
10100,
8,
198,
2,
220,
220,
220,
220,
1949,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
575,
796,
1441,
1100,
40664,
7,
35,
1404,
2969,
12599,
9,
1,
14,
52,
9419,
62,
4694,
62,
19895,
425,
62,
4626,
30487,
9,
3672,
9,
1,
30487,
9,
3672,
9,
1,
62,
51,
6465,
4943,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
14722,
796,
2835,
7,
5317,
11,
35138,
7,
56,
58,
45299,
16,
60,
4008,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
1366,
796,
1007,
3455,
7,
56,
58,
45299,
17,
25,
437,
12962,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
1441,
1366,
11,
23912,
1424,
198,
2,
220,
220,
220,
220,
4929,
11454,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
14643,
1472,
7,
19282,
448,
11,
11454,
11,
736,
40546,
35430,
35235,
3419,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
10951,
7203,
1639,
743,
423,
664,
39591,
428,
4049,
780,
345,
4398,
470,
15680,
262,
6831,
1865,
19570,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
10951,
7203,
23433,
2491,
4321,
62,
1229,
81,
3419,
717,
19570,
198,
2,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
10951,
7203,
1639,
743,
635,
423,
2984,
15803,
262,
1438,
286,
262,
27039,
19570,
198,
2,
220,
220,
220,
220,
886,
198,
2,
886,
198
] | 2.479101 | 1,914 |
"""
CurveWarpingSRSFSpace(knots, quadweights = get_quad_weights(knots))
Space of SRSFs of curve warpings on knots `knots`. Points are represented by vectors
in ambient space (ℝ^(N+1) if there are `N` knots). Given quadrature weights are used
to compute inner products.
"""
struct CurveWarpingSRSFSpace{TK<:AbstractVector,TW<:AbstractVector} <: AbstractManifold{ℝ}
knots::TK
quadweights::TW
end
function CurveWarpingSRSFSpace(knots::AbstractVector)
ws = get_quad_weights(knots)
return CurveWarpingSRSFSpace(knots, ws)
end
function manifold_dimension(M::CurveWarpingSRSFSpace)
return length(M.knots)
end
function representation_size(M::CurveWarpingSRSFSpace)
return (length(M.knots) + 1,)
end
function isapprox(M::CurveWarpingSRSFSpace, p, q; kwargs...)
return isapprox(distance(M, p, q), 0; kwargs...)
end
function isapprox(M::CurveWarpingSRSFSpace, p, X, Y; kwargs...)
return isapprox(X, Y; kwargs...)
end
"""
inner_ambient(M::CurveWarpingSRSFSpace, p, q)
Inner product in ambient space of points `p` and `q` on `M`. Takes into account quadrature
weights.
"""
function inner_ambient(M::CurveWarpingSRSFSpace, p, q)
return sum(M.quadweights .* p .* q)
end
function distance(M::CurveWarpingSRSFSpace, p, q)
pmq = p - q
ppq = p + q
return 2 * atan(sqrt(inner_ambient(M, pmq, pmq)), sqrt(inner_ambient(M, ppq, ppq)))
end
function embed!(::CurveWarpingSRSFSpace, q, p)
copyto!(q, p)
return q
end
function embed!(M::CurveWarpingSRSFSpace, Y, p, X)
copyto!(Y, X)
return Y
end
function project!(M::CurveWarpingSRSFSpace, q, p)
scale = sqrt(inner_ambient(M, p, p))
q .= p ./ scale
return q
end
function project!(M::CurveWarpingSRSFSpace, Y, p, X)
Y .= X .- inner_ambient(M, p, X) .* p
return Y
end
function get_quad_weights(nodes::AbstractRange)
n = length(nodes)
dt = 1 / (n - 1)
return dt / 2 .* [1; [2 for i in 1:(n - 2)]; 1]
# not that precise for some reason
#=if n % 2 == 1
# Simpson's rule
return dt / 3 .* [1; [i % 2 == 0 ? 2 : 4 for i in 1:(n - 2)]; 1]
else
# trapezoidal rule
return dt / 2 .* [1; [2 for i in 1:(n - 2)]; 1]
end=#
# Simpson's 3/8
#n%3 == 1 || error("argument mod 3 must be 1")
#return 3dt/8 .* [1; [i%3==0 ? 2 : 3 for i in 1:n-2]; 1]
end
function exp!(M::CurveWarpingSRSFSpace, q, p, X)
θ = norm(M, p, X)
q .= cos(θ) .* p .+ Manifolds.usinc(θ) .* X
return q
end
function log!(M::CurveWarpingSRSFSpace, X, p, q)
cosθ = clamp(inner_ambient(M, p, q), -1, 1)
θ = acos(cosθ)
X .= (q .- cosθ .* p) ./ Manifolds.usinc(θ)
return project!(M, X, p, X)
end
function inner(M::CurveWarpingSRSFSpace, p, X, Y)
return inner_ambient(M, X, Y)
end
function vector_transport_to!(M::CurveWarpingSRSFSpace, Y, p, X, q, ::ParallelTransport)
copyto!(Y, X)
X_pq = log(M, p, q)
X1 = norm(M, p, X_pq)
if X1 > 0
sum_pq = p + q
factor = 2 * inner_ambient(M, X, q) / inner_ambient(M, sum_pq, sum_pq)
Y .-= factor .* sum_pq
end
return Y
end
function zero_vector!(M::CurveWarpingSRSFSpace, X, p)
fill!(X, 0)
return X
end
const CurveWarpingSRSFGroup{TCWS<:CurveWarpingSRSFSpace} =
GroupManifold{ℝ,TCWS,WarpingCompositionOperation}
function CurveWarpingSRSFGroup(cws::CurveWarpingSRSFSpace)
return GroupManifold(cws, WarpingCompositionOperation())
end
function identity_element(cwg::CurveWarpingSRSFGroup)
return ones(length(cwg.manifold.knots))
end
function identity_element(cwg::CurveWarpingSRSFGroup, a)
return ones(eltype(a), length(cwg.manifold.knots))
end
function inv(cwg::CurveWarpingSRSFGroup, p)
cws = CurveWarpingSpace(cwg.manifold.knots)
pinv = inv(CurveWarpingGroup(cws), reverse_srsf(cws, p))
return srsf(cws, pinv)
end
inv(::CurveWarpingSRSFGroup, p::Identity{WarpingCompositionOperation}) = p
function compose(cwg::CurveWarpingSRSFGroup, p1, p2)
knots = cwg.manifold.knots
cws = CurveWarpingSpace(knots)
p1inv = reverse_srsf(cws, p1)
p2w = make_warping(cws, p2)
p2warped = map(t -> p2w(p1inv(t)), knots)
return p2warped .* p1
end
function compose(cwg::CurveWarpingSRSFGroup, p1::Identity{WarpingCompositionOperation}, p2)
return p2
end
function compose(cwg::CurveWarpingSRSFGroup, p1, p2::Identity{WarpingCompositionOperation})
return p1
end
function compose(
cwg::CurveWarpingSRSFGroup,
p1::Identity{WarpingCompositionOperation},
p2::Identity{WarpingCompositionOperation},
)
return p1
end
"""
CurveWarpingSRSFAction(M::AbstractManifold, p, cwg::CurveWarpingSRSFGroup)
Space of left actions of the group of SRSFs of curve warpings `cwg` on the manifold `M`
of TSRVFs of curves at point `p`.
"""
struct CurveWarpingSRSFAction{TM<:AbstractManifold,TP,TCWG<:CurveWarpingSRSFGroup} <:
AbstractGroupAction{LeftAction}
manifold::TM
point::TP
cwg::TCWG
end
function Manifolds.g_manifold(A::CurveWarpingSRSFAction)
return A.manifold
end
function Manifolds.base_group(A::CurveWarpingSRSFAction)
return A.cwg
end
function apply!(A::CurveWarpingSRSFAction{<:DiscretizedCurves}, q, a, p)
itp = make_interpolant(A.manifold, p)
a_rev = reverse_srsf(CurveWarpingSpace(A.cwg.manifold.knots), a)
ts = map(a_rev, A.cwg.manifold.knots)
rep_size = representation_size(A.manifold.manifold)
for (i, t) in zip(get_iterator(A.manifold), ts)
copyto!(_write(A.manifold, rep_size, q, i), itp(t) * a[i])
end
return q
end
function apply!(
A::CurveWarpingSRSFAction{<:DiscretizedCurves},
q,
::Identity{WarpingCompositionOperation},
p,
)
copyto!(q, p)
return q
end
function Manifolds.inverse_apply(A::CurveWarpingSRSFAction, a, p)
inva = inv(base_group(A), a)
return apply(A, inva, p)
end
function optimal_alignment(A::CurveWarpingSRSFAction, p, q)
M = A.manifold
return pairwise_optimal_warping(M, M, p, q, A.point)[1]
end
"""
karcher_mean_amplitude(A::CurveWarpingSRSFAction, ps::Vector)
Calculate the Karcher mean of amplitudes of given functions `ps` in SRVF form under
the action `A`.
Roughly follows Algorithm 2 from https://arxiv.org/abs/1103.3817.
"""
function karcher_mean_amplitude(
A::CurveWarpingSRSFAction,
ps::Vector;
throw_on_divergence=false,
progress_update=(x...) -> nothing,
max_iter=100,
)
M = A.manifold
N = length(ps)
cur_ps = ps
cur_increment = 42.0
prev_increment = 42.0
num_iterations = 0
meanp = project(M, (1.0 / N) * (sum(embed(M, p) for p in cur_ps)))
# find intial candidate for the mean
min_i = 1
norm_min_i = Inf
for i in 1:N
cur_norm = distance(M, meanp, cur_ps[i])
if cur_norm < norm_min_i
min_i = i
norm_min_i = cur_norm
end
end
curμp = cur_ps[min_i]
initial_increment = cur_increment
while cur_increment > 1e-6 && num_iterations < max_iter
warpings = [pairwise_optimal_warping(M, M, curμp, p, A.point) for p in cur_ps]
aligned_ps = [apply(A, warpings[i][1], cur_ps[i]) for i in 1:N]
mean_aligned_ps = project(M, (1.0 / N) * (sum(embed(M, p) for p in aligned_ps)))
prev_increment = cur_increment
cur_increment = distance(M, mean_aligned_ps, curμp)
progress_update(cur_increment, num_iterations, max_iter)
if num_iterations == 0
initial_increment = cur_increment
elseif cur_increment > 1.5 * prev_increment
if throw_on_divergence
error("Divergent mean amplitude calculation.")
else
break
end
end
cur_ps = aligned_ps
curμp = mean_aligned_ps
num_iterations += 1
end
progress_update(:finish)
return curμp
end
"""
phase_amplitude_separation(A::CurveWarpingSRSFAction, ps::Vector)
Perform phase-amplitude separation of given functions in SRVF form.
Returns mean amplitude, phases and amplitudes.
Implements alignment from Section 3.4 of https://arxiv.org/abs/1103.3817.
"""
function phase_amplitude_separation(A::CurveWarpingSRSFAction, ps::Vector)
μp = karcher_mean_amplitude(A, ps)
# TODO: use other mean functions?
a = center_of_orbit(A, ps, μp)
M = A.manifold
p̃ = apply(A, a, μp)
γs = [pairwise_optimal_warping(M, M, p̃, p, A.point)[1] for p in ps]
aligned_ps = [apply(A, γs[i], ps[i]) for i in 1:length(ps)]
return (p̃, γs, aligned_ps)
end
| [
37811,
198,
220,
220,
220,
46300,
54,
5117,
278,
50,
6998,
37,
14106,
7,
74,
1662,
82,
11,
15094,
43775,
796,
651,
62,
47003,
62,
43775,
7,
74,
1662,
82,
4008,
198,
198,
14106,
286,
311,
6998,
42388,
286,
12133,
25825,
654,
319,
33620,
4600,
74,
1662,
82,
44646,
11045,
389,
7997,
416,
30104,
198,
259,
25237,
2272,
357,
158,
226,
251,
61,
7,
45,
10,
16,
8,
611,
612,
389,
4600,
45,
63,
33620,
737,
11259,
15094,
81,
1300,
19590,
389,
973,
198,
1462,
24061,
8434,
3186,
13,
198,
37811,
198,
7249,
46300,
54,
5117,
278,
50,
6998,
37,
14106,
90,
51,
42,
27,
25,
23839,
38469,
11,
34551,
27,
25,
23839,
38469,
92,
1279,
25,
27741,
5124,
361,
727,
90,
158,
226,
251,
92,
198,
220,
220,
220,
33620,
3712,
51,
42,
198,
220,
220,
220,
15094,
43775,
3712,
34551,
198,
437,
198,
198,
8818,
46300,
54,
5117,
278,
50,
6998,
37,
14106,
7,
74,
1662,
82,
3712,
23839,
38469,
8,
198,
220,
220,
220,
266,
82,
796,
651,
62,
47003,
62,
43775,
7,
74,
1662,
82,
8,
198,
220,
220,
220,
1441,
46300,
54,
5117,
278,
50,
6998,
37,
14106,
7,
74,
1662,
82,
11,
266,
82,
8,
198,
437,
198,
198,
8818,
48048,
62,
46156,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
8,
198,
220,
220,
220,
1441,
4129,
7,
44,
13,
74,
1662,
82,
8,
198,
437,
198,
198,
8818,
10552,
62,
7857,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
8,
198,
220,
220,
220,
1441,
357,
13664,
7,
44,
13,
74,
1662,
82,
8,
1343,
352,
35751,
198,
437,
198,
198,
8818,
318,
1324,
13907,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
279,
11,
10662,
26,
479,
86,
22046,
23029,
198,
220,
220,
220,
1441,
318,
1324,
13907,
7,
30246,
7,
44,
11,
279,
11,
10662,
828,
657,
26,
479,
86,
22046,
23029,
198,
437,
198,
198,
8818,
318,
1324,
13907,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
279,
11,
1395,
11,
575,
26,
479,
86,
22046,
23029,
198,
220,
220,
220,
1441,
318,
1324,
13907,
7,
55,
11,
575,
26,
479,
86,
22046,
23029,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
8434,
62,
4131,
1153,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
279,
11,
10662,
8,
198,
198,
818,
1008,
1720,
287,
25237,
2272,
286,
2173,
4600,
79,
63,
290,
4600,
80,
63,
319,
4600,
44,
44646,
33687,
656,
1848,
15094,
81,
1300,
198,
43775,
13,
198,
37811,
198,
8818,
8434,
62,
4131,
1153,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
279,
11,
10662,
8,
198,
220,
220,
220,
1441,
2160,
7,
44,
13,
47003,
43775,
764,
9,
279,
764,
9,
10662,
8,
198,
437,
198,
198,
8818,
5253,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
279,
11,
10662,
8,
198,
220,
220,
220,
9114,
80,
796,
279,
532,
10662,
198,
220,
220,
220,
9788,
80,
796,
279,
1343,
10662,
198,
220,
220,
220,
1441,
362,
1635,
379,
272,
7,
31166,
17034,
7,
5083,
62,
4131,
1153,
7,
44,
11,
9114,
80,
11,
9114,
80,
36911,
19862,
17034,
7,
5083,
62,
4131,
1153,
7,
44,
11,
9788,
80,
11,
9788,
80,
22305,
198,
437,
198,
198,
8818,
11525,
0,
7,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
10662,
11,
279,
8,
198,
220,
220,
220,
4866,
1462,
0,
7,
80,
11,
279,
8,
198,
220,
220,
220,
1441,
10662,
198,
437,
198,
198,
8818,
11525,
0,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
575,
11,
279,
11,
1395,
8,
198,
220,
220,
220,
4866,
1462,
0,
7,
56,
11,
1395,
8,
198,
220,
220,
220,
1441,
575,
198,
437,
198,
198,
8818,
1628,
0,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
10662,
11,
279,
8,
198,
220,
220,
220,
5046,
796,
19862,
17034,
7,
5083,
62,
4131,
1153,
7,
44,
11,
279,
11,
279,
4008,
198,
220,
220,
220,
10662,
764,
28,
279,
24457,
5046,
198,
220,
220,
220,
1441,
10662,
198,
437,
198,
198,
8818,
1628,
0,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
575,
11,
279,
11,
1395,
8,
198,
220,
220,
220,
575,
764,
28,
1395,
764,
12,
8434,
62,
4131,
1153,
7,
44,
11,
279,
11,
1395,
8,
764,
9,
279,
198,
220,
220,
220,
1441,
575,
198,
437,
198,
198,
8818,
651,
62,
47003,
62,
43775,
7,
77,
4147,
3712,
23839,
17257,
8,
198,
220,
220,
220,
299,
796,
4129,
7,
77,
4147,
8,
198,
220,
220,
220,
288,
83,
796,
352,
1220,
357,
77,
532,
352,
8,
198,
220,
220,
220,
1441,
288,
83,
1220,
362,
764,
9,
685,
16,
26,
685,
17,
329,
1312,
287,
352,
37498,
77,
532,
362,
8,
11208,
352,
60,
198,
220,
220,
220,
1303,
407,
326,
7141,
329,
617,
1738,
198,
220,
220,
220,
1303,
28,
361,
299,
4064,
362,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
20531,
338,
3896,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
288,
83,
1220,
513,
764,
9,
685,
16,
26,
685,
72,
4064,
362,
6624,
657,
5633,
362,
1058,
604,
329,
1312,
287,
352,
37498,
77,
532,
362,
8,
11208,
352,
60,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
1291,
46057,
47502,
3896,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
288,
83,
1220,
362,
764,
9,
685,
16,
26,
685,
17,
329,
1312,
287,
352,
37498,
77,
532,
362,
8,
11208,
352,
60,
198,
220,
220,
220,
886,
46249,
628,
220,
220,
220,
1303,
20531,
338,
513,
14,
23,
198,
220,
220,
220,
1303,
77,
4,
18,
6624,
352,
8614,
4049,
7203,
49140,
953,
513,
1276,
307,
352,
4943,
198,
220,
220,
220,
1303,
7783,
513,
28664,
14,
23,
764,
9,
685,
16,
26,
685,
72,
4,
18,
855,
15,
5633,
362,
1058,
513,
329,
1312,
287,
352,
25,
77,
12,
17,
11208,
352,
60,
198,
437,
198,
198,
8818,
1033,
0,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
10662,
11,
279,
11,
1395,
8,
198,
220,
220,
220,
7377,
116,
796,
2593,
7,
44,
11,
279,
11,
1395,
8,
198,
220,
220,
220,
10662,
764,
28,
8615,
7,
138,
116,
8,
764,
9,
279,
764,
10,
1869,
361,
10119,
13,
385,
1939,
7,
138,
116,
8,
764,
9,
1395,
198,
220,
220,
220,
1441,
10662,
198,
437,
198,
198,
8818,
2604,
0,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
1395,
11,
279,
11,
10662,
8,
198,
220,
220,
220,
8615,
138,
116,
796,
29405,
7,
5083,
62,
4131,
1153,
7,
44,
11,
279,
11,
10662,
828,
532,
16,
11,
352,
8,
198,
220,
220,
220,
7377,
116,
796,
936,
418,
7,
6966,
138,
116,
8,
198,
220,
220,
220,
1395,
764,
28,
357,
80,
764,
12,
8615,
138,
116,
764,
9,
279,
8,
24457,
1869,
361,
10119,
13,
385,
1939,
7,
138,
116,
8,
198,
220,
220,
220,
1441,
1628,
0,
7,
44,
11,
1395,
11,
279,
11,
1395,
8,
198,
437,
198,
198,
8818,
8434,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
279,
11,
1395,
11,
575,
8,
198,
220,
220,
220,
1441,
8434,
62,
4131,
1153,
7,
44,
11,
1395,
11,
575,
8,
198,
437,
198,
198,
8818,
15879,
62,
7645,
634,
62,
1462,
0,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
575,
11,
279,
11,
1395,
11,
10662,
11,
7904,
10044,
29363,
8291,
634,
8,
198,
220,
220,
220,
4866,
1462,
0,
7,
56,
11,
1395,
8,
198,
220,
220,
220,
1395,
62,
79,
80,
796,
2604,
7,
44,
11,
279,
11,
10662,
8,
198,
220,
220,
220,
1395,
16,
796,
2593,
7,
44,
11,
279,
11,
1395,
62,
79,
80,
8,
198,
220,
220,
220,
611,
1395,
16,
1875,
657,
198,
220,
220,
220,
220,
220,
220,
220,
2160,
62,
79,
80,
796,
279,
1343,
10662,
198,
220,
220,
220,
220,
220,
220,
220,
5766,
796,
362,
1635,
8434,
62,
4131,
1153,
7,
44,
11,
1395,
11,
10662,
8,
1220,
8434,
62,
4131,
1153,
7,
44,
11,
2160,
62,
79,
80,
11,
2160,
62,
79,
80,
8,
198,
220,
220,
220,
220,
220,
220,
220,
575,
764,
12,
28,
5766,
764,
9,
2160,
62,
79,
80,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
575,
198,
437,
198,
198,
8818,
6632,
62,
31364,
0,
7,
44,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
11,
1395,
11,
279,
8,
198,
220,
220,
220,
6070,
0,
7,
55,
11,
657,
8,
198,
220,
220,
220,
1441,
1395,
198,
437,
198,
198,
9979,
46300,
54,
5117,
278,
50,
6998,
37,
13247,
90,
4825,
19416,
27,
25,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
92,
796,
198,
220,
220,
220,
4912,
5124,
361,
727,
90,
158,
226,
251,
11,
4825,
19416,
11,
54,
5117,
278,
5377,
9150,
32180,
92,
198,
198,
8818,
46300,
54,
5117,
278,
50,
6998,
37,
13247,
7,
66,
18504,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
14106,
8,
198,
220,
220,
220,
1441,
4912,
5124,
361,
727,
7,
66,
18504,
11,
1810,
13886,
5377,
9150,
32180,
28955,
198,
437,
198,
198,
8818,
5369,
62,
30854,
7,
66,
86,
70,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
13247,
8,
198,
220,
220,
220,
1441,
3392,
7,
13664,
7,
66,
86,
70,
13,
805,
361,
727,
13,
74,
1662,
82,
4008,
198,
437,
198,
8818,
5369,
62,
30854,
7,
66,
86,
70,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
13247,
11,
257,
8,
198,
220,
220,
220,
1441,
3392,
7,
417,
4906,
7,
64,
828,
4129,
7,
66,
86,
70,
13,
805,
361,
727,
13,
74,
1662,
82,
4008,
198,
437,
198,
198,
8818,
800,
7,
66,
86,
70,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
13247,
11,
279,
8,
198,
220,
220,
220,
269,
18504,
796,
46300,
54,
5117,
278,
14106,
7,
66,
86,
70,
13,
805,
361,
727,
13,
74,
1662,
82,
8,
198,
220,
220,
220,
6757,
85,
796,
800,
7,
26628,
303,
54,
5117,
278,
13247,
7,
66,
18504,
828,
9575,
62,
82,
3808,
69,
7,
66,
18504,
11,
279,
4008,
198,
220,
220,
220,
1441,
264,
3808,
69,
7,
66,
18504,
11,
6757,
85,
8,
198,
437,
198,
16340,
7,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
13247,
11,
279,
3712,
7390,
26858,
90,
54,
5117,
278,
5377,
9150,
32180,
30072,
796,
279,
198,
198,
8818,
36664,
7,
66,
86,
70,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
13247,
11,
279,
16,
11,
279,
17,
8,
198,
220,
220,
220,
33620,
796,
269,
86,
70,
13,
805,
361,
727,
13,
74,
1662,
82,
198,
220,
220,
220,
269,
18504,
796,
46300,
54,
5117,
278,
14106,
7,
74,
1662,
82,
8,
198,
220,
220,
220,
279,
16,
16340,
796,
9575,
62,
82,
3808,
69,
7,
66,
18504,
11,
279,
16,
8,
198,
220,
220,
220,
279,
17,
86,
796,
787,
62,
86,
5117,
278,
7,
66,
18504,
11,
279,
17,
8,
198,
220,
220,
220,
279,
17,
86,
5117,
276,
796,
3975,
7,
83,
4613,
279,
17,
86,
7,
79,
16,
16340,
7,
83,
36911,
33620,
8,
198,
220,
220,
220,
1441,
279,
17,
86,
5117,
276,
764,
9,
279,
16,
198,
437,
198,
8818,
36664,
7,
66,
86,
70,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
13247,
11,
279,
16,
3712,
7390,
26858,
90,
54,
5117,
278,
5377,
9150,
32180,
5512,
279,
17,
8,
198,
220,
220,
220,
1441,
279,
17,
198,
437,
198,
8818,
36664,
7,
66,
86,
70,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
13247,
11,
279,
16,
11,
279,
17,
3712,
7390,
26858,
90,
54,
5117,
278,
5377,
9150,
32180,
30072,
198,
220,
220,
220,
1441,
279,
16,
198,
437,
198,
8818,
36664,
7,
198,
220,
220,
220,
269,
86,
70,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
13247,
11,
198,
220,
220,
220,
279,
16,
3712,
7390,
26858,
90,
54,
5117,
278,
5377,
9150,
32180,
5512,
198,
220,
220,
220,
279,
17,
3712,
7390,
26858,
90,
54,
5117,
278,
5377,
9150,
32180,
5512,
198,
8,
198,
220,
220,
220,
1441,
279,
16,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
46300,
54,
5117,
278,
50,
6998,
7708,
596,
7,
44,
3712,
23839,
5124,
361,
727,
11,
279,
11,
269,
86,
70,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
37,
13247,
8,
198,
198,
14106,
286,
1364,
4028,
286,
262,
1448,
286,
311,
6998,
42388,
286,
12133,
25825,
654,
4600,
66,
86,
70,
63,
319,
262,
48048,
4600,
44,
63,
198,
1659,
309,
12562,
53,
42388,
286,
23759,
379,
966,
4600,
79,
44646,
198,
37811,
198,
7249,
46300,
54,
5117,
278,
50,
6998,
7708,
596,
90,
15972,
27,
25,
23839,
5124,
361,
727,
11,
7250,
11,
4825,
54,
38,
27,
25,
26628,
303,
54,
5117,
278,
50,
6998,
37,
13247,
92,
1279,
25,
198,
220,
220,
220,
220,
220,
220,
27741,
13247,
12502,
90,
18819,
12502,
92,
198,
220,
220,
220,
48048,
3712,
15972,
198,
220,
220,
220,
966,
3712,
7250,
198,
220,
220,
220,
269,
86,
70,
3712,
4825,
54,
38,
198,
437,
198,
198,
8818,
1869,
361,
10119,
13,
70,
62,
805,
361,
727,
7,
32,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
7708,
596,
8,
198,
220,
220,
220,
1441,
317,
13,
805,
361,
727,
198,
437,
198,
198,
8818,
1869,
361,
10119,
13,
8692,
62,
8094,
7,
32,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
7708,
596,
8,
198,
220,
220,
220,
1441,
317,
13,
66,
86,
70,
198,
437,
198,
198,
8818,
4174,
0,
7,
32,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
7708,
596,
90,
27,
25,
15642,
1186,
1143,
26628,
1158,
5512,
10662,
11,
257,
11,
279,
8,
198,
220,
220,
220,
340,
79,
796,
787,
62,
3849,
16104,
415,
7,
32,
13,
805,
361,
727,
11,
279,
8,
198,
220,
220,
220,
257,
62,
18218,
796,
9575,
62,
82,
3808,
69,
7,
26628,
303,
54,
5117,
278,
14106,
7,
32,
13,
66,
86,
70,
13,
805,
361,
727,
13,
74,
1662,
82,
828,
257,
8,
198,
220,
220,
220,
40379,
796,
3975,
7,
64,
62,
18218,
11,
317,
13,
66,
86,
70,
13,
805,
361,
727,
13,
74,
1662,
82,
8,
198,
220,
220,
220,
1128,
62,
7857,
796,
10552,
62,
7857,
7,
32,
13,
805,
361,
727,
13,
805,
361,
727,
8,
198,
220,
220,
220,
329,
357,
72,
11,
256,
8,
287,
19974,
7,
1136,
62,
48727,
7,
32,
13,
805,
361,
727,
828,
40379,
8,
198,
220,
220,
220,
220,
220,
220,
220,
4866,
1462,
0,
28264,
13564,
7,
32,
13,
805,
361,
727,
11,
1128,
62,
7857,
11,
10662,
11,
1312,
828,
340,
79,
7,
83,
8,
1635,
257,
58,
72,
12962,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
10662,
198,
437,
198,
198,
8818,
4174,
0,
7,
198,
220,
220,
220,
317,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
7708,
596,
90,
27,
25,
15642,
1186,
1143,
26628,
1158,
5512,
198,
220,
220,
220,
10662,
11,
198,
220,
220,
220,
7904,
7390,
26858,
90,
54,
5117,
278,
5377,
9150,
32180,
5512,
198,
220,
220,
220,
279,
11,
198,
8,
198,
220,
220,
220,
4866,
1462,
0,
7,
80,
11,
279,
8,
198,
220,
220,
220,
1441,
10662,
198,
437,
198,
198,
8818,
1869,
361,
10119,
13,
259,
4399,
62,
39014,
7,
32,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
7708,
596,
11,
257,
11,
279,
8,
198,
220,
220,
220,
800,
64,
796,
800,
7,
8692,
62,
8094,
7,
32,
828,
257,
8,
198,
220,
220,
220,
1441,
4174,
7,
32,
11,
800,
64,
11,
279,
8,
198,
437,
198,
198,
8818,
16586,
62,
282,
16747,
7,
32,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
7708,
596,
11,
279,
11,
10662,
8,
198,
220,
220,
220,
337,
796,
317,
13,
805,
361,
727,
198,
220,
220,
220,
1441,
5166,
3083,
62,
8738,
4402,
62,
86,
5117,
278,
7,
44,
11,
337,
11,
279,
11,
10662,
11,
317,
13,
4122,
38381,
16,
60,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
479,
283,
2044,
62,
32604,
62,
321,
489,
3984,
7,
32,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
7708,
596,
11,
26692,
3712,
38469,
8,
198,
198,
9771,
3129,
378,
262,
9375,
2044,
1612,
286,
12306,
10455,
286,
1813,
5499,
4600,
862,
63,
287,
16808,
53,
37,
1296,
739,
198,
1169,
2223,
4600,
32,
44646,
198,
198,
49,
619,
306,
5679,
978,
42289,
362,
422,
3740,
1378,
283,
87,
452,
13,
2398,
14,
8937,
14,
11442,
18,
13,
2548,
1558,
13,
198,
37811,
198,
8818,
479,
283,
2044,
62,
32604,
62,
321,
489,
3984,
7,
198,
220,
220,
220,
317,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
7708,
596,
11,
198,
220,
220,
220,
26692,
3712,
38469,
26,
198,
220,
220,
220,
3714,
62,
261,
62,
67,
1428,
12745,
28,
9562,
11,
198,
220,
220,
220,
4371,
62,
19119,
16193,
87,
23029,
4613,
2147,
11,
198,
220,
220,
220,
3509,
62,
2676,
28,
3064,
11,
198,
8,
198,
220,
220,
220,
337,
796,
317,
13,
805,
361,
727,
198,
220,
220,
220,
399,
796,
4129,
7,
862,
8,
198,
220,
220,
220,
1090,
62,
862,
796,
26692,
198,
220,
220,
220,
1090,
62,
24988,
434,
796,
5433,
13,
15,
198,
220,
220,
220,
8654,
62,
24988,
434,
796,
5433,
13,
15,
198,
220,
220,
220,
997,
62,
2676,
602,
796,
657,
198,
220,
220,
220,
1612,
79,
796,
1628,
7,
44,
11,
357,
16,
13,
15,
1220,
399,
8,
1635,
357,
16345,
7,
20521,
7,
44,
11,
279,
8,
329,
279,
287,
1090,
62,
862,
22305,
628,
220,
220,
220,
1303,
1064,
493,
498,
4540,
329,
262,
1612,
198,
220,
220,
220,
949,
62,
72,
796,
352,
198,
220,
220,
220,
2593,
62,
1084,
62,
72,
796,
4806,
198,
220,
220,
220,
329,
1312,
287,
352,
25,
45,
198,
220,
220,
220,
220,
220,
220,
220,
1090,
62,
27237,
796,
5253,
7,
44,
11,
1612,
79,
11,
1090,
62,
862,
58,
72,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
611,
1090,
62,
27237,
1279,
2593,
62,
1084,
62,
72,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
949,
62,
72,
796,
1312,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2593,
62,
1084,
62,
72,
796,
1090,
62,
27237,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1090,
34703,
79,
796,
1090,
62,
862,
58,
1084,
62,
72,
60,
198,
220,
220,
220,
4238,
62,
24988,
434,
796,
1090,
62,
24988,
434,
198,
220,
220,
220,
981,
1090,
62,
24988,
434,
1875,
352,
68,
12,
21,
11405,
997,
62,
2676,
602,
1279,
3509,
62,
2676,
198,
220,
220,
220,
220,
220,
220,
220,
25825,
654,
796,
685,
24874,
3083,
62,
8738,
4402,
62,
86,
5117,
278,
7,
44,
11,
337,
11,
1090,
34703,
79,
11,
279,
11,
317,
13,
4122,
8,
329,
279,
287,
1090,
62,
862,
60,
628,
220,
220,
220,
220,
220,
220,
220,
19874,
62,
862,
796,
685,
39014,
7,
32,
11,
25825,
654,
58,
72,
7131,
16,
4357,
1090,
62,
862,
58,
72,
12962,
329,
1312,
287,
352,
25,
45,
60,
198,
220,
220,
220,
220,
220,
220,
220,
1612,
62,
41634,
62,
862,
796,
1628,
7,
44,
11,
357,
16,
13,
15,
1220,
399,
8,
1635,
357,
16345,
7,
20521,
7,
44,
11,
279,
8,
329,
279,
287,
19874,
62,
862,
22305,
198,
220,
220,
220,
220,
220,
220,
220,
8654,
62,
24988,
434,
796,
1090,
62,
24988,
434,
198,
220,
220,
220,
220,
220,
220,
220,
1090,
62,
24988,
434,
796,
5253,
7,
44,
11,
1612,
62,
41634,
62,
862,
11,
1090,
34703,
79,
8,
198,
220,
220,
220,
220,
220,
220,
220,
4371,
62,
19119,
7,
22019,
62,
24988,
434,
11,
997,
62,
2676,
602,
11,
3509,
62,
2676,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
997,
62,
2676,
602,
6624,
657,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4238,
62,
24988,
434,
796,
1090,
62,
24988,
434,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
361,
1090,
62,
24988,
434,
1875,
352,
13,
20,
1635,
8654,
62,
24988,
434,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
3714,
62,
261,
62,
67,
1428,
12745,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4049,
7203,
35,
1428,
6783,
1612,
37188,
17952,
19570,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2270,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
1090,
62,
862,
796,
19874,
62,
862,
198,
220,
220,
220,
220,
220,
220,
220,
1090,
34703,
79,
796,
1612,
62,
41634,
62,
862,
198,
220,
220,
220,
220,
220,
220,
220,
997,
62,
2676,
602,
15853,
352,
198,
220,
220,
220,
886,
198,
220,
220,
220,
4371,
62,
19119,
7,
25,
15643,
680,
8,
628,
220,
220,
220,
1441,
1090,
34703,
79,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
7108,
62,
321,
489,
3984,
62,
25512,
341,
7,
32,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
7708,
596,
11,
26692,
3712,
38469,
8,
198,
198,
5990,
687,
7108,
12,
321,
489,
3984,
14139,
286,
1813,
5499,
287,
16808,
53,
37,
1296,
13,
198,
35561,
1612,
37188,
11,
21164,
290,
12306,
10455,
13,
198,
198,
3546,
1154,
902,
19114,
422,
7275,
513,
13,
19,
286,
3740,
1378,
283,
87,
452,
13,
2398,
14,
8937,
14,
11442,
18,
13,
2548,
1558,
13,
198,
37811,
198,
8818,
7108,
62,
321,
489,
3984,
62,
25512,
341,
7,
32,
3712,
26628,
303,
54,
5117,
278,
50,
6998,
7708,
596,
11,
26692,
3712,
38469,
8,
198,
220,
220,
220,
18919,
79,
796,
479,
283,
2044,
62,
32604,
62,
321,
489,
3984,
7,
32,
11,
26692,
8,
198,
220,
220,
220,
1303,
16926,
46,
25,
779,
584,
1612,
5499,
30,
198,
220,
220,
220,
257,
796,
3641,
62,
1659,
62,
42594,
7,
32,
11,
26692,
11,
18919,
79,
8,
198,
220,
220,
220,
337,
796,
317,
13,
805,
361,
727,
198,
220,
220,
220,
279,
136,
225,
796,
4174,
7,
32,
11,
257,
11,
18919,
79,
8,
198,
220,
220,
220,
7377,
111,
82,
796,
685,
24874,
3083,
62,
8738,
4402,
62,
86,
5117,
278,
7,
44,
11,
337,
11,
279,
136,
225,
11,
279,
11,
317,
13,
4122,
38381,
16,
60,
329,
279,
287,
26692,
60,
198,
220,
220,
220,
19874,
62,
862,
796,
685,
39014,
7,
32,
11,
7377,
111,
82,
58,
72,
4357,
26692,
58,
72,
12962,
329,
1312,
287,
352,
25,
13664,
7,
862,
15437,
198,
220,
220,
220,
1441,
357,
79,
136,
225,
11,
7377,
111,
82,
11,
19874,
62,
862,
8,
198,
437,
198
] | 2.168286 | 3,910 |
module CanvasWebIO
using WebIO, JSExpr, Observables
export Canvas, addmovable!, addclickable!, addstatic!
mutable struct Canvas
w::WebIO.Scope
size::Array{Int64, 1}
objects::Array{WebIO.Node, 1}
getter::Dict
id::String
handler::Observables.Observable
selection::Observables.Observable
synced::Bool # synced=true => julia listeners called on mousemove, not just drop
end
function Canvas(size::Array{Int64,1}, synced=false)
w = Scope(imports=["/pkg/CanvasWebIO/helpers.js"])
handler = Observable(w, "handler", ["id", 0, 0])
selection = Observable(w, "selection", "id")
getter = Dict()
id = WebIO.newid("canvas")
on(selection) do val
val
end
on(handler) do val
selection[] = val[1]
if val[1] in keys(getter)
getter[val[1]][] = Int.(floor.(val[2:3]))
else
println("Failed to assign value $(val[2:3]) to $(val[1])")
end
end
Canvas(w, size, Array{WebIO.Node,1}(), getter, id, handler, selection, synced)
end
function Canvas()
Canvas([800,800])
end
function Canvas(synced::Bool)
Canvas([800,800], synced)
end
function Base.getindex(canvas::Canvas, i)
canvas.getter[i]
end
function (canvas::Canvas)()
# js function setp sets the position of the object named name to the position of the mouse
# returns the [draggable, xpos, ypos] where draggable is whether the object was movable,
# and xpos,ypos the new position of the object
#
# Transform parser from https://stackoverflow.com/a/17838403
canvas_events = Dict()
handler = canvas.handler
synced = canvas.synced
canvas_events["mousemove"] = @js function(event)
event.preventDefault()
event.stopPropagation()
@var name = document.getElementById($(canvas.id)).getAttribute("data-selected")
@var pos
if name!=""
pos = setp(event, name)
if(pos[0]) #is dragged
document.getElementById($(canvas.id)).setAttribute("is-dragged", true)
if($synced)
$handler[] = [name, Math.round(pos[1]), Math.round(pos[2])]
end
end
end
end
canvas_events["mouseup"] = @js function(event)
event.preventDefault()
event.stopPropagation()
console.log("canvas click")
@var name = document.getElementById($(canvas.id)).getAttribute("data-selected")
@var pos
if name!=""
pos = setp(event, name)
if document.getElementById($(canvas.id)).getAttribute("is-dragged")=="true"
$handler[] = [name, parseFloat(pos[1]), parseFloat(pos[2])]
document.getElementById(name).style.stroke = "none"
document.getElementById($(canvas.id)).setAttribute("data-selected", "")
document.getElementById($(canvas.id)).setAttribute("is-dragged", false)
end
end
end
canvas.w(dom"svg:svg[id = $(canvas.id),
height = $(canvas.size[1]),
width = $(canvas.size[2])]"(
canvas.objects...,
attributes = Dict("data-selected" => "",
"is-dragged" => false),
events = canvas_events))
end
"""
addclickable!(canvas::Canvas, svg::WebIO.Node)
Adds a clickable (as in, can be clicked but not moved) object to the canvas based on the svg template. If the template has an id, this will be given to the canvas object, and the object will be associated with the id as a string (canvas[id] accesses the associated observable etc). If the template has no id, one will be generated. Note that the stroke property will be overwritten.
"""
function addclickable!(canvas::Canvas, svg::WebIO.Node)
attr = svg.props[:attributes]
children = svg.children
if "id" in keys(attr)
id = attr["id"]
else
id = WebIO.newid("svg")
end
selection = canvas.selection
clickable_events = Dict()
clickable_events["click"] = @js function(event)
name = document.getElementById($(canvas.id)).getAttribute("data-selected")
#selected_obj
if name == this.id
this.style.stroke = "none"
document.getElementById($(canvas.id)).setAttribute("data-selected", "")
else
if name != ""
selected_obj = document.getElementById(name)
selected_obj.style.stroke = "none"
end
this.style.stroke = "green" #Change this later
this.style.strokeWidth = 2 #Change this later
document.getElementById($(canvas.id)).setAttribute("data-selected", this.id)
$selection[] = this.id
end
end
push!(canvas.objects,
Node(svg.instanceof, children..., attributes=attr, events=clickable_events))
end
"""
addmovable!(canvas::Canvas, svg::WebIO.Node, lock=" ")
Adds a movable object to the canvas based on the svg template. If the template has an id, this will be given to the canvas object, and the object will be associated with the id as a string (canvas[id] accesses the associated observable etc). If the template has no id, one will be generated. Note that the stroke property will be overwritten.
The optional lock argument allows locking of an axis. Setting lock="x" will lock the movable's x value, so it can only be moved up and down. Similarly, lock="y" will only permit movements to the left and right.
"""
function addmovable!(canvas::Canvas, svg::WebIO.Node, lock=" ")
attr = svg.props[:attributes]
if svg.instanceof.tag!=:g
newattr = Dict()
if "id" in keys(attr)
newattr["id"] = attr["id"]
else
newattr["id"] = WebIO.newid("svg")
end
attr["id"] = WebIO.newid("subsvg")
if haskey(attr, "x") && haskey(attr, "y") #Rectangle etc
newattr["transform"] = "translate($(attr["x"]),$(attr["y"]))"
attr["x"] = "$(-parse(attr["width"])/2)"
attr["y"] = "$(-parse(attr["height"])/2)"
elseif haskey(attr, "cx") && haskey(attr, "cy") #Circle
newattr["transform"] = "translate($(attr["cx"]),$(attr["cy"]))"
attr["cx"] = "0.0"
attr["cy"] = "0.0"
else
newattr["transform"] = "translate($(attr["cx"]),$(attr["cy"]))" #undefined object
end
return addmovable!(canvas, dom"svg:g"(svg, attributes=newattr), lock)
end
if :style in keys(svg.props)
style = svg.props[:style]
else
style = Dict()
end
children = svg.children
if "id" in keys(attr)
id = attr["id"]
else
id = WebIO.newid("svg")
attr["id"] = id
end
attr["data-lock"] = lock
if svg.instanceof.tag==:g
coo = [0.0, 0.0]
try
coo .= parse.(Float64, match(r"translate\((.*?),(.*?)\)",
attr["transform"]).captures)
catch
println("Failed to get position of $id, setting default")
end
pos = Observable(canvas.w, id, coo)
else
error("Only <g> objects allowed")
end
push!(pos.listeners, (x)->(x))
canvas.getter[id] = pos
handler = canvas.handler
attr["draggable"] = "true"
style[:cursor] = "move"
movable_events = Dict()
movable_events["mousedown"] = @js function(event)
event.stopPropagation()
event.preventDefault()
console.log("clicking", this.id)
@var name = document.getElementById($(canvas.id)).getAttribute("data-selected")
@var pos
if name == ""
this.style.stroke = "red" #Change this later
this.style.strokeWidth = 2 #Change this later
document.getElementById($(canvas.id)).setAttribute("data-selected", this.id)
else
selected_obj = document.getElementById(name)
selected_obj.style.stroke = "none"
pos = setp(event,name)
if(pos[0]) #is dragged
$handler[] = [name, pos[1], pos[2]]
end
document.getElementById($(canvas.id)).setAttribute("data-selected", "")
document.getElementById($(canvas.id)).setAttribute("is-dragged", false)
end
end
node = Node(svg.instanceof, children..., attributes=attr, style=style, events=movable_events)
push!(canvas.objects, node)
node
end
"""
addstatic!(canvas::Canvas, svg::WebIO.Node)
Adds the svg object directly to the canvas.
"""
function addstatic!(canvas::Canvas, svg::WebIO.Node)
push!(canvas.objects, svg)
end
"""
setindex_(canvas::Canvas, pos, i::String)
Sets the position of the object i to pos on the javascript side.
"""
function setindex_(canvas::Canvas, pos, i::String)
evaljs(canvas.w, js""" setp_nonevent($pos, $i)""")
end
function Base.setindex!(canvas::Canvas, val, i::String)
setindex_(canvas::Canvas, val, i)
canvas[i][] = val
end
end
| [
21412,
1680,
11017,
13908,
9399,
198,
198,
3500,
5313,
9399,
11,
26755,
3109,
1050,
11,
19243,
2977,
198,
198,
39344,
1680,
11017,
11,
751,
76,
21985,
28265,
751,
12976,
540,
28265,
751,
12708,
0,
198,
198,
76,
18187,
2878,
1680,
11017,
198,
220,
220,
220,
266,
3712,
13908,
9399,
13,
43642,
198,
220,
220,
220,
2546,
3712,
19182,
90,
5317,
2414,
11,
352,
92,
198,
220,
220,
220,
5563,
3712,
19182,
90,
13908,
9399,
13,
19667,
11,
352,
92,
198,
220,
220,
220,
651,
353,
3712,
35,
713,
198,
220,
220,
220,
4686,
3712,
10100,
198,
220,
220,
220,
21360,
3712,
31310,
712,
2977,
13,
31310,
712,
540,
198,
220,
220,
220,
6356,
3712,
31310,
712,
2977,
13,
31310,
712,
540,
198,
220,
220,
220,
6171,
771,
3712,
33,
970,
1303,
6171,
771,
28,
7942,
5218,
474,
43640,
22054,
1444,
319,
10211,
21084,
11,
407,
655,
4268,
198,
437,
198,
198,
8818,
1680,
11017,
7,
7857,
3712,
19182,
90,
5317,
2414,
11,
16,
5512,
6171,
771,
28,
9562,
8,
198,
220,
220,
220,
266,
796,
41063,
7,
320,
3742,
28,
14692,
14,
35339,
14,
6090,
11017,
13908,
9399,
14,
16794,
364,
13,
8457,
8973,
8,
198,
220,
220,
220,
21360,
796,
19243,
540,
7,
86,
11,
366,
30281,
1600,
14631,
312,
1600,
657,
11,
657,
12962,
198,
220,
220,
220,
6356,
796,
19243,
540,
7,
86,
11,
366,
49283,
1600,
366,
312,
4943,
198,
220,
220,
220,
651,
353,
796,
360,
713,
3419,
198,
220,
220,
220,
4686,
796,
5313,
9399,
13,
3605,
312,
7203,
5171,
11017,
4943,
198,
220,
220,
220,
319,
7,
49283,
8,
466,
1188,
198,
220,
220,
220,
220,
220,
220,
220,
1188,
198,
220,
220,
220,
886,
198,
220,
220,
220,
319,
7,
30281,
8,
466,
1188,
198,
220,
220,
220,
220,
220,
220,
220,
6356,
21737,
796,
1188,
58,
16,
60,
198,
220,
220,
220,
220,
220,
220,
220,
611,
1188,
58,
16,
60,
287,
8251,
7,
1136,
353,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
651,
353,
58,
2100,
58,
16,
60,
7131,
60,
796,
2558,
12195,
28300,
12195,
2100,
58,
17,
25,
18,
60,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
44872,
7203,
37,
6255,
284,
8333,
1988,
29568,
2100,
58,
17,
25,
18,
12962,
284,
29568,
2100,
58,
16,
12962,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1680,
11017,
7,
86,
11,
2546,
11,
15690,
90,
13908,
9399,
13,
19667,
11,
16,
92,
22784,
651,
353,
11,
4686,
11,
21360,
11,
6356,
11,
6171,
771,
8,
198,
437,
198,
198,
8818,
1680,
11017,
3419,
198,
220,
220,
220,
1680,
11017,
26933,
7410,
11,
7410,
12962,
198,
437,
198,
198,
8818,
1680,
11017,
7,
28869,
771,
3712,
33,
970,
8,
198,
220,
220,
220,
1680,
11017,
26933,
7410,
11,
7410,
4357,
6171,
771,
8,
198,
437,
198,
198,
8818,
7308,
13,
1136,
9630,
7,
5171,
11017,
3712,
6090,
11017,
11,
1312,
8,
198,
220,
220,
220,
21978,
13,
1136,
353,
58,
72,
60,
198,
437,
198,
198,
8818,
357,
5171,
11017,
3712,
6090,
11017,
8,
3419,
628,
220,
220,
220,
1303,
44804,
2163,
900,
79,
5621,
262,
2292,
286,
262,
2134,
3706,
1438,
284,
262,
2292,
286,
262,
10211,
198,
220,
220,
220,
1303,
5860,
262,
685,
7109,
9460,
540,
11,
2124,
1930,
11,
331,
1930,
60,
810,
6715,
70,
540,
318,
1771,
262,
2134,
373,
1409,
540,
11,
198,
220,
220,
220,
1303,
290,
2124,
1930,
11,
88,
1930,
262,
649,
2292,
286,
262,
2134,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
26981,
30751,
422,
3740,
1378,
25558,
2502,
11125,
13,
785,
14,
64,
14,
23188,
2548,
31552,
628,
220,
220,
220,
21978,
62,
31534,
796,
360,
713,
3419,
628,
220,
220,
220,
21360,
796,
21978,
13,
30281,
198,
220,
220,
220,
6171,
771,
220,
796,
21978,
13,
28869,
771,
628,
220,
220,
220,
21978,
62,
31534,
14692,
35888,
21084,
8973,
220,
796,
2488,
8457,
2163,
7,
15596,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1785,
13,
3866,
1151,
19463,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
1785,
13,
11338,
24331,
363,
341,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
7785,
1438,
796,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
1136,
33682,
7203,
7890,
12,
34213,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
7785,
1426,
198,
220,
220,
220,
220,
220,
220,
220,
611,
1438,
0,
33151,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1426,
796,
900,
79,
7,
15596,
11,
1438,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
7,
1930,
58,
15,
12962,
1303,
271,
17901,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
2617,
33682,
7203,
271,
12,
7109,
14655,
1600,
2081,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
16763,
28869,
771,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
720,
30281,
21737,
796,
685,
3672,
11,
16320,
13,
744,
7,
1930,
58,
16,
46570,
16320,
13,
744,
7,
1930,
58,
17,
12962,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
21978,
62,
31534,
14692,
35888,
929,
8973,
796,
2488,
8457,
2163,
7,
15596,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1785,
13,
3866,
1151,
19463,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
1785,
13,
11338,
24331,
363,
341,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
8624,
13,
6404,
7203,
5171,
11017,
3904,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
7785,
1438,
796,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
1136,
33682,
7203,
7890,
12,
34213,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
7785,
1426,
198,
220,
220,
220,
220,
220,
220,
220,
611,
1438,
0,
33151,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1426,
796,
900,
79,
7,
15596,
11,
1438,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
1136,
33682,
7203,
271,
12,
7109,
14655,
4943,
855,
1,
7942,
1,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
720,
30281,
21737,
796,
685,
3672,
11,
21136,
43879,
7,
1930,
58,
16,
46570,
21136,
43879,
7,
1930,
58,
17,
12962,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3188,
13,
1136,
20180,
48364,
7,
3672,
737,
7635,
13,
30757,
796,
366,
23108,
1,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
2617,
33682,
7203,
7890,
12,
34213,
1600,
366,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
2617,
33682,
7203,
271,
12,
7109,
14655,
1600,
3991,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
21978,
13,
86,
7,
3438,
1,
21370,
70,
25,
21370,
70,
58,
312,
796,
29568,
5171,
11017,
13,
312,
828,
198,
220,
220,
220,
220,
220,
220,
220,
6001,
796,
29568,
5171,
11017,
13,
7857,
58,
16,
46570,
198,
220,
220,
220,
220,
220,
220,
220,
9647,
796,
29568,
5171,
11017,
13,
7857,
58,
17,
12962,
60,
18109,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
21978,
13,
48205,
986,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
12608,
796,
360,
713,
7203,
7890,
12,
34213,
1,
5218,
366,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
271,
12,
7109,
14655,
1,
5218,
3991,
828,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2995,
796,
21978,
62,
31534,
4008,
198,
437,
198,
198,
37811,
198,
2860,
12976,
540,
0,
7,
5171,
11017,
3712,
6090,
11017,
11,
38487,
70,
3712,
13908,
9399,
13,
19667,
8,
198,
198,
46245,
257,
3904,
540,
357,
292,
287,
11,
460,
307,
28384,
475,
407,
3888,
8,
2134,
284,
262,
21978,
1912,
319,
262,
38487,
70,
11055,
13,
1002,
262,
11055,
468,
281,
4686,
11,
428,
481,
307,
1813,
284,
262,
21978,
2134,
11,
290,
262,
2134,
481,
307,
3917,
351,
262,
4686,
355,
257,
4731,
357,
5171,
11017,
58,
312,
60,
1895,
274,
262,
3917,
42550,
3503,
737,
1002,
262,
11055,
468,
645,
4686,
11,
530,
481,
307,
7560,
13,
5740,
326,
262,
14000,
3119,
481,
307,
6993,
9108,
13,
198,
37811,
198,
8818,
751,
12976,
540,
0,
7,
5171,
11017,
3712,
6090,
11017,
11,
38487,
70,
3712,
13908,
9399,
13,
19667,
8,
198,
220,
220,
220,
708,
81,
796,
38487,
70,
13,
1676,
862,
58,
25,
1078,
7657,
60,
198,
220,
220,
220,
1751,
796,
38487,
70,
13,
17197,
198,
220,
220,
220,
611,
366,
312,
1,
287,
8251,
7,
35226,
8,
198,
220,
220,
220,
220,
220,
220,
220,
4686,
796,
708,
81,
14692,
312,
8973,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
4686,
796,
5313,
9399,
13,
3605,
312,
7203,
21370,
70,
4943,
198,
220,
220,
220,
886,
198,
220,
220,
220,
6356,
796,
21978,
13,
49283,
198,
220,
220,
220,
3904,
540,
62,
31534,
796,
360,
713,
3419,
198,
220,
220,
220,
3904,
540,
62,
31534,
14692,
12976,
8973,
220,
796,
2488,
8457,
2163,
7,
15596,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1438,
796,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
1136,
33682,
7203,
7890,
12,
34213,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
34213,
62,
26801,
198,
220,
220,
220,
220,
220,
220,
220,
611,
1438,
6624,
428,
13,
312,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
428,
13,
7635,
13,
30757,
796,
366,
23108,
1,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
2617,
33682,
7203,
7890,
12,
34213,
1600,
366,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
1438,
14512,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6163,
62,
26801,
796,
3188,
13,
1136,
20180,
48364,
7,
3672,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6163,
62,
26801,
13,
7635,
13,
30757,
796,
366,
23108,
1,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
428,
13,
7635,
13,
30757,
796,
366,
14809,
1,
1303,
19400,
428,
1568,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
428,
13,
7635,
13,
30757,
30916,
796,
362,
1303,
19400,
428,
1568,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
2617,
33682,
7203,
7890,
12,
34213,
1600,
428,
13,
312,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
720,
49283,
21737,
796,
428,
13,
312,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
4574,
0,
7,
5171,
11017,
13,
48205,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
19081,
7,
21370,
70,
13,
39098,
1659,
11,
1751,
986,
11,
12608,
28,
35226,
11,
2995,
28,
12976,
540,
62,
31534,
4008,
198,
437,
198,
37811,
198,
2860,
76,
21985,
0,
7,
5171,
11017,
3712,
6090,
11017,
11,
38487,
70,
3712,
13908,
9399,
13,
19667,
11,
5793,
2625,
366,
8,
198,
198,
46245,
257,
1409,
540,
2134,
284,
262,
21978,
1912,
319,
262,
38487,
70,
11055,
13,
1002,
262,
11055,
468,
281,
4686,
11,
428,
481,
307,
1813,
284,
262,
21978,
2134,
11,
290,
262,
2134,
481,
307,
3917,
351,
262,
4686,
355,
257,
4731,
357,
5171,
11017,
58,
312,
60,
1895,
274,
262,
3917,
42550,
3503,
737,
1002,
262,
11055,
468,
645,
4686,
11,
530,
481,
307,
7560,
13,
5740,
326,
262,
14000,
3119,
481,
307,
6993,
9108,
13,
198,
198,
464,
11902,
5793,
4578,
3578,
22656,
286,
281,
16488,
13,
25700,
5793,
2625,
87,
1,
481,
5793,
262,
1409,
540,
338,
2124,
1988,
11,
523,
340,
460,
691,
307,
3888,
510,
290,
866,
13,
15298,
11,
5793,
2625,
88,
1,
481,
691,
8749,
8650,
284,
262,
1364,
290,
826,
13,
198,
37811,
198,
8818,
751,
76,
21985,
0,
7,
5171,
11017,
3712,
6090,
11017,
11,
38487,
70,
3712,
13908,
9399,
13,
19667,
11,
5793,
2625,
366,
8,
198,
220,
220,
220,
708,
81,
796,
38487,
70,
13,
1676,
862,
58,
25,
1078,
7657,
60,
198,
220,
220,
220,
611,
38487,
70,
13,
39098,
1659,
13,
12985,
0,
28,
25,
70,
198,
220,
220,
220,
220,
220,
220,
220,
649,
35226,
796,
360,
713,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
611,
366,
312,
1,
287,
8251,
7,
35226,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
35226,
14692,
312,
8973,
796,
708,
81,
14692,
312,
8973,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
35226,
14692,
312,
8973,
796,
5313,
9399,
13,
3605,
312,
7203,
21370,
70,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
708,
81,
14692,
312,
8973,
796,
5313,
9399,
13,
3605,
312,
7203,
7266,
21370,
70,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
611,
468,
2539,
7,
35226,
11,
366,
87,
4943,
11405,
468,
2539,
7,
35226,
11,
366,
88,
4943,
1303,
45474,
9248,
3503,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
35226,
14692,
35636,
8973,
796,
366,
7645,
17660,
16763,
7,
35226,
14692,
87,
8973,
828,
3,
7,
35226,
14692,
88,
8973,
4008,
1,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
708,
81,
14692,
87,
8973,
796,
17971,
32590,
29572,
7,
35226,
14692,
10394,
8973,
20679,
17,
16725,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
708,
81,
14692,
88,
8973,
796,
17971,
32590,
29572,
7,
35226,
14692,
17015,
8973,
20679,
17,
16725,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
361,
468,
2539,
7,
35226,
11,
366,
66,
87,
4943,
11405,
468,
2539,
7,
35226,
11,
366,
948,
4943,
1303,
31560,
293,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
35226,
14692,
35636,
8973,
796,
366,
7645,
17660,
16763,
7,
35226,
14692,
66,
87,
8973,
828,
3,
7,
35226,
14692,
948,
8973,
4008,
1,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
708,
81,
14692,
66,
87,
8973,
796,
366,
15,
13,
15,
1,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
708,
81,
14692,
948,
8973,
796,
366,
15,
13,
15,
1,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
35226,
14692,
35636,
8973,
796,
366,
7645,
17660,
16763,
7,
35226,
14692,
66,
87,
8973,
828,
3,
7,
35226,
14692,
948,
8973,
4008,
1,
1303,
917,
18156,
2134,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
751,
76,
21985,
0,
7,
5171,
11017,
11,
2401,
1,
21370,
70,
25,
70,
18109,
21370,
70,
11,
12608,
28,
3605,
35226,
828,
5793,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
611,
1058,
7635,
287,
8251,
7,
21370,
70,
13,
1676,
862,
8,
198,
220,
220,
220,
220,
220,
220,
220,
3918,
796,
38487,
70,
13,
1676,
862,
58,
25,
7635,
60,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
3918,
796,
360,
713,
3419,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1751,
796,
38487,
70,
13,
17197,
198,
220,
220,
220,
611,
366,
312,
1,
287,
8251,
7,
35226,
8,
198,
220,
220,
220,
220,
220,
220,
220,
4686,
796,
708,
81,
14692,
312,
8973,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
4686,
796,
5313,
9399,
13,
3605,
312,
7203,
21370,
70,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
708,
81,
14692,
312,
8973,
796,
4686,
198,
220,
220,
220,
886,
198,
220,
220,
220,
708,
81,
14692,
7890,
12,
5354,
8973,
796,
5793,
198,
220,
220,
220,
611,
38487,
70,
13,
39098,
1659,
13,
12985,
855,
25,
70,
198,
220,
220,
220,
220,
220,
220,
220,
763,
78,
796,
685,
15,
13,
15,
11,
657,
13,
15,
60,
198,
220,
220,
220,
220,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
763,
78,
764,
28,
21136,
12195,
43879,
2414,
11,
2872,
7,
81,
1,
7645,
17660,
59,
19510,
15885,
33924,
7,
15885,
10091,
22725,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
708,
81,
14692,
35636,
8973,
737,
27144,
942,
8,
198,
220,
220,
220,
220,
220,
220,
220,
4929,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
44872,
7203,
37,
6255,
284,
651,
2292,
286,
720,
312,
11,
4634,
4277,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
1426,
796,
19243,
540,
7,
5171,
11017,
13,
86,
11,
4686,
11,
763,
78,
8,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
4049,
7203,
10049,
1279,
70,
29,
5563,
3142,
4943,
198,
220,
220,
220,
886,
628,
220,
220,
220,
4574,
0,
7,
1930,
13,
4868,
36014,
11,
357,
87,
8,
3784,
7,
87,
4008,
198,
220,
220,
220,
21978,
13,
1136,
353,
58,
312,
60,
796,
1426,
628,
220,
220,
220,
21360,
796,
21978,
13,
30281,
198,
220,
220,
220,
708,
81,
14692,
7109,
9460,
540,
8973,
796,
366,
7942,
1,
198,
220,
220,
220,
3918,
58,
25,
66,
21471,
60,
796,
366,
21084,
1,
198,
220,
220,
220,
1409,
540,
62,
31534,
796,
360,
713,
3419,
628,
220,
220,
220,
1409,
540,
62,
31534,
14692,
76,
29997,
593,
8973,
220,
796,
2488,
8457,
2163,
7,
15596,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1785,
13,
11338,
24331,
363,
341,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
1785,
13,
3866,
1151,
19463,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
8624,
13,
6404,
7203,
565,
7958,
1600,
428,
13,
312,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
7785,
1438,
796,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
1136,
33682,
7203,
7890,
12,
34213,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
7785,
1426,
198,
220,
220,
220,
220,
220,
220,
220,
611,
1438,
6624,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
428,
13,
7635,
13,
30757,
796,
366,
445,
1,
1303,
19400,
428,
1568,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
428,
13,
7635,
13,
30757,
30916,
796,
362,
1303,
19400,
428,
1568,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
2617,
33682,
7203,
7890,
12,
34213,
1600,
428,
13,
312,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6163,
62,
26801,
796,
3188,
13,
1136,
20180,
48364,
7,
3672,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6163,
62,
26801,
13,
7635,
13,
30757,
796,
366,
23108,
1,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1426,
796,
900,
79,
7,
15596,
11,
3672,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
7,
1930,
58,
15,
12962,
1303,
271,
17901,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
720,
30281,
21737,
796,
685,
3672,
11,
1426,
58,
16,
4357,
1426,
58,
17,
11907,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
2617,
33682,
7203,
7890,
12,
34213,
1600,
366,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3188,
13,
1136,
20180,
48364,
16763,
7,
5171,
11017,
13,
312,
29720,
2617,
33682,
7203,
271,
12,
7109,
14655,
1600,
3991,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
10139,
796,
19081,
7,
21370,
70,
13,
39098,
1659,
11,
1751,
986,
11,
12608,
28,
35226,
11,
3918,
28,
7635,
11,
2995,
28,
76,
21985,
62,
31534,
8,
198,
220,
220,
220,
4574,
0,
7,
5171,
11017,
13,
48205,
11,
10139,
8,
198,
220,
220,
220,
10139,
198,
437,
198,
198,
37811,
198,
2860,
12708,
0,
7,
5171,
11017,
3712,
6090,
11017,
11,
38487,
70,
3712,
13908,
9399,
13,
19667,
8,
198,
198,
46245,
262,
38487,
70,
2134,
3264,
284,
262,
21978,
13,
198,
37811,
198,
8818,
751,
12708,
0,
7,
5171,
11017,
3712,
6090,
11017,
11,
38487,
70,
3712,
13908,
9399,
13,
19667,
8,
198,
220,
220,
220,
4574,
0,
7,
5171,
11017,
13,
48205,
11,
38487,
70,
8,
198,
437,
198,
198,
37811,
198,
2617,
9630,
41052,
5171,
11017,
3712,
6090,
11017,
11,
1426,
11,
1312,
3712,
10100,
8,
198,
198,
50,
1039,
262,
2292,
286,
262,
2134,
1312,
284,
1426,
319,
262,
44575,
1735,
13,
198,
37811,
198,
8818,
900,
9630,
41052,
5171,
11017,
3712,
6090,
11017,
11,
1426,
11,
1312,
3712,
10100,
8,
198,
220,
220,
220,
5418,
8457,
7,
5171,
11017,
13,
86,
11,
44804,
37811,
900,
79,
62,
23108,
1151,
16763,
1930,
11,
720,
72,
8,
15931,
4943,
198,
437,
198,
198,
8818,
7308,
13,
2617,
9630,
0,
7,
5171,
11017,
3712,
6090,
11017,
11,
1188,
11,
1312,
3712,
10100,
8,
198,
220,
220,
220,
900,
9630,
41052,
5171,
11017,
3712,
6090,
11017,
11,
1188,
11,
1312,
8,
198,
220,
220,
220,
21978,
58,
72,
7131,
60,
796,
1188,
198,
437,
198,
198,
437,
198
] | 2.248195 | 4,017 |
module Fatou
using SyntaxTree,Reduce,LaTeXStrings,Requires,Base.Threads
# This file is part of Fatou.jl. It is licensed under the MIT license
# Copyright (C) 2017 Michael Reed
export fatou, juliafill, mandelbrot, newton, basin, plot
"""
Fatou.Define(E::Any; # primary map, (z, c) -> F
Q::Expr = :(abs2(z)), # escape criterion, (z, c) -> Q
C::Expr = :((angle(z)/(2π))*n^p)# coloring, (z, n=iter., p=exp.) -> C
∂ = π/2, # Array{Float64,1} # Bounds, [x(a),x(b),y(a),y(b)]
n::Integer = 176, # horizontal grid points
N::Integer = 35, # max. iterations
ϵ::Number = 4, # basin ϵ-Limit criterion
iter::Bool = false, # toggle iteration mode
p::Number = 0, # iteration color exponent
newt::Bool = false, # toggle Newton mode
m::Number = 0, # Newton multiplicity factor
O::String = F, # original Newton map
mandel::Bool= false, # toggle Mandelbrot mode
seed::Number= 0.0+0.0im, # Mandelbrot seed value
x0 = nothing, # orbit starting point
orbit::Int = 0, # orbit cobweb depth
depth::Int = 1, # depth of function composition
cmap::String= "", # imshow color map
plane::Bool = false, # convert input disk to half-plane
disk::Bool = false) # convert output half-plane to disk
`Define` the metadata for a `Fatou.FilledSet`.
"""
struct Define{FT<:Function,QT<:Function,CT<:Function,M,N,P,D}
E::Any # input expression
F::FT # primary map
Q::QT # escape criterion
C::CT # complex fixed point coloring
∂::Array{Float64,1} # bounds
n::UInt16 # number of grid points
N::UInt8 # number of iterations
ϵ::Float64 # epsilon Limit criterion
iter::Bool # toggle iteration mode
p::Float64 # iteration color exponent
newt::Bool # toggle Newton mode
m::Number # newton multiplicity factor
mandel::Bool # toggle Mandelbrot mode
seed::Number # Mandelbrot seed value
x0 # orbit starting point
orbit::Int # orbit cobweb depth
depth::Int # depth of function composition
cmap::String # imshow color map
plane::Bool # convert input disk to half-plane
disk::Bool # convert output half-plane to disk
function Define(E;
Q=:(abs2(z)),
C=:((angle(z)/(2π))*n^p),
∂=π/2,
n::Integer=176,
N::Integer=35,
ϵ::Number=4,
iter::Bool=false,
p::Number=0,
newt::Bool=false,
m::Number=0,
mandel::Bool=false,
seed::Number=0.0+0.0im,
x0=nothing,
orbit::Int=0,
depth::Int=1,
cmap::String="",
plane::Bool=false,
disk::Bool=false)
!(typeof(∂) <: Array) && (∂ = [-float(∂),∂,-∂,∂])
length(∂) == 2 && (∂ = [∂[1],∂[2],∂[1],∂[2]])
!newt ? (f = genfun(E,[:z,:c]); q = genfun(Q,[:z,:c])) :
(f = genfun(newton_raphson(E,m),[:z,:c]); q = genfun(Expr(:call,:abs,E),[:z,:c]))
c = genfun(C,[:z,:n,:p])
e = typeof(E) == String ? parse(E) : E
return new{typeof(f),typeof(q),typeof(c),mandel,newt,plane,disk}(e,f,q,c,convert(Array{Float64,1},∂),UInt16(n),UInt8(N),float(ϵ),iter,float(p),newt,m,mandel,seed,x0,orbit,depth,cmap,plane,disk)
end
end
"""
Fatou.FilledSet(::Fatou.Define)
Compute the `Fatou.FilledSet` set using `Fatou.Define`.
"""
struct FilledSet{FT,QT,CT,M,N,P,D}
meta::Define{FT,QT,CT,M,N,P,D}
set::Matrix{Complex{Float64}}
iter::Matrix{UInt8}
mix::Matrix{Float64}
function FilledSet{FT,QT,CT,M,N,P,D}(K::Define{FT,QT,CT,M,N,P,D}) where {FT,QT,CT,M,N,P,D}
(i,s) = Compute(K)
return new{FT,QT,CT,M,N,P,D}(K,s,i,broadcast(K.C,s,broadcast(float,i./K.N),K.p))
end
end
"""
fatou(::Fatou.Define)
Compute the `Fatou.FilledSet` set using `Fatou.Define`.
# Examples
```Julia
julia> fatou(K)
```
"""
fatou(K::Define{FT,QT,CT,M,N,P,D}) where {FT,QT,CT,M,N,P,D} = FilledSet{FT,QT,CT,M,N,P,D}(K)
"""
juliafill(::Expr; # primary map, (z, c) -> F
Q::Expr = :(abs2(z)), # escape criterion, (z, c) -> Q
C::Expr = :((angle(z)/(2π))*n^p)# coloring, (z, n=iter., p=exp.) -> C
∂ = π/2, # Array{Float64,1} # Bounds, [x(a),x(b),y(a),y(b)]
n::Integer = 176, # horizontal grid points
N::Integer = 35, # max. iterations
ϵ::Number = 4, # basin ϵ-Limit criterion
iter::Bool = false, # toggle iteration mode
p::Number = 0, # iteration color exponent
x0 = nothing, # orbit starting point
orbit::Int = 0, # orbit cobweb depth
depth::Int = 1, # depth of function composition
cmap::String= "", # imshow color map
plane::Bool = false, # convert input disk to half-plane
disk::Bool = false) # convert output half-plane to disk
`Define` filled Julia basin in `Fatou`
# Exmaples
```Julia
julia> juliafill("z^2-0.06+0.67im",∂=[-1.5,1.5,-1,1],N=80,n=1501,cmap="RdGy")
```
"""
function juliafill(E;
Q=:(abs2(z)),
C=:((angle(z)/(2π))*n^p),
∂=π/2,
n::Integer=176,
N::Integer=35,
ϵ::Number=4,
iter::Bool=false,
p::Number=0,
newt::Bool=false,
m::Number=0,
x0=nothing,
orbit::Int=0,
depth::Int=1,
cmap::String="",
plane::Bool=false,
disk::Bool=false)
return Define(E,Q=Q,C=C,∂=∂,n=n,N=N,ϵ=ϵ,iter=iter,p=p,newt=newt,m=m,x0=x0,orbit=orbit,depth=depth,cmap=cmap,plane=plane,disk=disk)
end
"""
mandelbrot(::Expr; # primary map, (z, c) -> F
Q::Expr = :(abs2(z)), # escape criterion, (z, c) -> Q
C::Expr = :(exp(-abs(z))*n^p), # coloring, (z, n=iter., p=exp.) -> C
∂ = π/2, # Array{Float64,1} # Bounds, [x(a),x(b),y(a),y(b)]
n::Integer = 176, # horizontal grid points
N::Integer = 35, # max. iterations
ϵ::Number = 4, # basin ϵ-Limit criterion
iter::Bool = false, # toggle iteration mode
p::Number = 0, # iteration color exponent
m::Number = 0, # Newton multiplicity factor
seed::Number= 0.0+0.0im, # Mandelbrot seed value
x0 = nothing, # orbit starting point
orbit::Int = 0, # orbit cobweb depth
depth::Int = 1, # depth of function composition
cmap::String= "", # imshow color map
plane::Bool = false, # convert input disk to half-plane
disk::Bool = false) # convert output half-plane to disk
`Define` Mandelbrot basin in `Fatou`
# Examples
```Julia
mandelbrot(:(z^2+c),n=800,N=20,∂=[-1.91,0.51,-1.21,1.21],cmap="nipy_spectral")
```
"""
function mandelbrot(E;
Q=:(abs2(z)),
∂=π/2,
C=:(exp(-abs(z))*n^p),
n::Integer=176,
N::Integer=35,
ϵ::Number=4,
iter::Bool=false,
p::Number=0,
newt::Bool=false,
m::Number=0,
seed::Number=0.0+0.0im,
x0=nothing,
orbit::Int=0,
depth::Int=1,
cmap::String="",
plane::Bool=false,
disk::Bool=false)
m ≠ 0 && (newt = true)
return Define(E,Q=Q,C=C,∂=∂,n=n,N=N,ϵ=ϵ,iter=iter,p=p,newt=newt,m=m,mandel=true,seed=seed,x0=x0,orbit=orbit,depth=depth,cmap=cmap,plane=plane,disk=disk)
end
"""
newton(::Expr; # primary map, (z, c) -> F
C::Expr = :((angle(z)/(2π))*n^p)# coloring, (z, n=iter., p=exp.) -> C
∂ = π/2, # Array{Float64,1} # Bounds, [x(a),x(b),y(a),y(b)]
n::Integer = 176, # horizontal grid points
N::Integer = 35, # max. iterations
ϵ::Number = 4, # basin ϵ-Limit criterion
iter::Bool = false, # toggle iteration mode
p::Number = 0, # iteration color exponent
m::Number = 0, # Newton multiplicity factor
mandel::Bool= false, # toggle Mandelbrot mode
seed::Number= 0.0+0.0im, # Mandelbrot seed value
x0 = nothing, # orbit starting point
orbit::Int = 0, # orbit cobweb depth
depth::Int = 1, # depth of function composition
cmap::String= "", # imshow color map
plane::Bool = false, # convert input disk to half-plane
disk::Bool = false) # convert output half-plane to disk
`Define` Newton basin in `Fatou`
# Examples
```Julia
julia> newton("z^3-1",n=800,cmap="brg")
```
"""
function newton(E;
C=:((angle(z)/(2π))*n^p),
∂=π/2,
n::Integer=176,
N::Integer=35,
ϵ::Number=0.01,
iter::Bool=false,
p::Number=0,
m::Number=1,
mandel::Bool=false,
seed::Number=0.0+0.0im,
x0=nothing,
orbit::Int=0,
depth::Int=1,
cmap::String="",
plane::Bool=false,
disk::Bool=false)
return Define(E,C=C,∂=∂,n=n,N=N,ϵ=ϵ,iter=iter,p=p,newt=true,m=m,mandel=mandel,seed=seed,x0=x0,orbit=orbit,depth=depth,cmap=cmap,plane=plane,disk=disk)
end
# load additional functionality
include("internals.jl"); include("orbitplot.jl")
"""
basin(::Fatou.Define, ::Integer)
Output the `j`-th basin of `Fatou.Define` as LaTeX.
Each subsequent iteration of the Newton-Raphson method will yield a more complicated set.
# Examples
```Julia
julia> basin(newton("z^3-1"),2)
L"\$\\displaystyle D_2(\\epsilon) = \\left\\{z\\in\\mathbb{C}:\\left|\\,z - \\frac{\\left(z - \\frac{z^{3} - 1}{3 z^{2}}\\right)^{3} - 1}{3 \\left(z - \\frac{z^{3} - 1}{3 z^{2}}\\right)^{2}} - \\frac{z^{3} - 1}{3 z^{2}} - r_i\\,\\right|<\\epsilon,\\,\\forall r_i(\\,f(r_i)=0 )\\right\\}\$"
```
"""
basin(K::Define,j) = K.newt ? nrset(K.E,K.m,j) : jset(K.E,j)
plane(z::Complex) = (2z.re/(z.re^2+(1-z.im)^2))+im*(1-z.re^2-z.im^2)/(z.re^2+(1-z.im)^2)
disk(z::Complex) = (2z.re/(z.re^2+(1+z.im)^2))+im*(z.re^2+z.im^2-1)/(z.re^2+(1+z.im)^2)
# define function for computing orbit of a z0 input
function orbit(K::Define{FT,QT,CT,M,N,P,D},z0::Complex{Float64}) where {FT,QT,CT,M,N,P,D}
M ? (z = K.seed) : (z = P ? plane(z0) : z0)
zn = 0x00
while (N ? (K.Q(z,z0)::Float64>K.ϵ)::Bool : (K.Q(z,z0)::Float64<K.ϵ))::Bool && K.N>zn
z = K.F(z,z0)::Complex{Float64}
zn+=0x01
end; #end
# return the normalized argument of z or iteration count
return (zn::UInt8,(D ? disk(z) : z)::Complex{Float64})
end
"""
Compute(::Fatou.Define)::Union{Matrix{UInt8},Matrix{Float64}}
`Compute` the `Array` for `Fatou.FilledSet` as specefied by `Fatou.Define`.
"""
function Compute(K::Define{FT,QT,CT,M,N,D})::Tuple{Matrix{UInt8},Matrix{Complex{Float64}}} where {FT,QT,CT,M,N,D}
# generate coordinate grid
Kyn = round(UInt16,(K.∂[4]-K.∂[3])/(K.∂[2]-K.∂[1])*K.n)
x = range(K.∂[1]+0.0001,stop=K.∂[2],length=K.n)
y = range(K.∂[4],stop=K.∂[3],length=Kyn)
Z = x' .+ im*y # apply Newton-Orbit function element-wise to coordinate grid
(matU,matF) = (Array{UInt8,2}(undef,Kyn,K.n),Array{Complex{Float64},2}(undef,Kyn,K.n))
@time @threads for j = 1:length(y); for k = 1:length(x);
(matU[j,k],matF[j,k]) = orbit(K,Z[j,k])::Tuple{UInt8,Complex{Float64}}
end; end
return (matU,matF)
end
function __init__()
println("Fatou detected $(Threads.nthreads()) julia threads.")
@require PyPlot="d330b81b-6aea-500a-939a-2ce795aea3ee" include("pyplot.jl")
@require ImageInTerminal="d8c32880-2388-543b-8c61-d9f865259254" include("term.jl")
@require UnicodePlots="b8865327-cd53-5732-bb35-84acbb429228" include("uniplots.jl")
end
end # module
| [
21412,
12301,
280,
198,
3500,
26375,
897,
27660,
11,
7738,
7234,
11,
14772,
49568,
13290,
654,
11,
39618,
11,
14881,
13,
16818,
82,
198,
198,
2,
220,
220,
770,
2393,
318,
636,
286,
12301,
280,
13,
20362,
13,
632,
318,
11971,
739,
262,
17168,
5964,
198,
2,
220,
220,
15069,
357,
34,
8,
2177,
3899,
16105,
198,
198,
39344,
3735,
280,
11,
474,
43640,
20797,
11,
6855,
417,
7957,
83,
11,
649,
1122,
11,
34164,
11,
7110,
198,
198,
37811,
198,
220,
220,
220,
12301,
280,
13,
7469,
500,
7,
36,
3712,
7149,
26,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
4165,
3975,
11,
357,
89,
11,
269,
8,
4613,
376,
198,
220,
220,
220,
220,
220,
1195,
3712,
3109,
1050,
220,
220,
220,
220,
796,
36147,
8937,
17,
7,
89,
36911,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
6654,
34054,
11,
357,
89,
11,
269,
8,
4613,
1195,
198,
220,
220,
220,
220,
220,
327,
3712,
3109,
1050,
220,
220,
220,
220,
796,
1058,
19510,
9248,
7,
89,
20679,
7,
17,
46582,
4008,
9,
77,
61,
79,
8,
2,
33988,
11,
357,
89,
11,
299,
28,
2676,
1539,
279,
28,
11201,
2014,
4613,
327,
198,
220,
220,
220,
220,
220,
18872,
224,
220,
220,
220,
796,
18074,
222,
14,
17,
11,
1303,
15690,
90,
43879,
2414,
11,
16,
92,
220,
220,
220,
220,
220,
1303,
347,
3733,
11,
685,
87,
7,
64,
828,
87,
7,
65,
828,
88,
7,
64,
828,
88,
7,
65,
15437,
198,
220,
220,
220,
220,
220,
299,
3712,
46541,
220,
796,
26937,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
16021,
10706,
2173,
198,
220,
220,
220,
220,
220,
399,
3712,
46541,
220,
796,
3439,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
3509,
13,
34820,
198,
220,
220,
220,
220,
220,
18074,
113,
3712,
15057,
220,
220,
796,
604,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
34164,
18074,
113,
12,
39184,
34054,
198,
220,
220,
220,
220,
220,
11629,
3712,
33,
970,
220,
796,
3991,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
19846,
24415,
4235,
198,
220,
220,
220,
220,
220,
279,
3712,
15057,
220,
220,
796,
657,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
24415,
3124,
28622,
198,
220,
220,
220,
220,
220,
649,
83,
3712,
33,
970,
220,
796,
3991,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
19846,
17321,
4235,
198,
220,
220,
220,
220,
220,
285,
3712,
15057,
220,
220,
796,
657,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
17321,
15082,
8467,
5766,
198,
220,
220,
220,
220,
220,
440,
3712,
10100,
220,
220,
796,
376,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
2656,
17321,
3975,
198,
220,
220,
220,
220,
220,
6855,
417,
3712,
33,
970,
28,
3991,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
19846,
13314,
417,
7957,
83,
4235,
198,
220,
220,
220,
220,
220,
9403,
3712,
15057,
28,
657,
13,
15,
10,
15,
13,
15,
320,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
13314,
417,
7957,
83,
9403,
1988,
198,
220,
220,
220,
220,
220,
2124,
15,
220,
220,
220,
220,
220,
220,
220,
220,
220,
796,
2147,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
13066,
3599,
966,
198,
220,
220,
220,
220,
220,
13066,
3712,
5317,
220,
796,
657,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
13066,
22843,
12384,
6795,
198,
220,
220,
220,
220,
220,
6795,
3712,
5317,
220,
796,
352,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
6795,
286,
2163,
11742,
198,
220,
220,
220,
220,
220,
269,
8899,
3712,
10100,
28,
366,
1600,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
545,
12860,
3124,
3975,
198,
220,
220,
220,
220,
220,
6614,
3712,
33,
970,
796,
3991,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
10385,
5128,
11898,
284,
2063,
12,
14382,
198,
220,
220,
220,
220,
220,
11898,
3712,
33,
970,
220,
796,
3991,
8,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
10385,
5072,
2063,
12,
14382,
284,
11898,
198,
198,
63,
7469,
500,
63,
262,
20150,
329,
257,
4600,
33804,
280,
13,
37,
2967,
7248,
44646,
198,
37811,
198,
7249,
2896,
500,
90,
9792,
27,
25,
22203,
11,
48,
51,
27,
25,
22203,
11,
4177,
27,
25,
22203,
11,
44,
11,
45,
11,
47,
11,
35,
92,
198,
220,
220,
220,
412,
3712,
7149,
1303,
5128,
5408,
198,
220,
220,
220,
376,
3712,
9792,
1303,
4165,
3975,
198,
220,
220,
220,
1195,
3712,
48,
51,
1303,
6654,
34054,
198,
220,
220,
220,
327,
3712,
4177,
1303,
3716,
5969,
966,
33988,
198,
220,
220,
220,
18872,
224,
3712,
19182,
90,
43879,
2414,
11,
16,
92,
1303,
22303,
198,
220,
220,
220,
299,
3712,
52,
5317,
1433,
1303,
1271,
286,
10706,
2173,
198,
220,
220,
220,
399,
3712,
52,
5317,
23,
1303,
1271,
286,
34820,
198,
220,
220,
220,
18074,
113,
3712,
43879,
2414,
1303,
304,
862,
33576,
27272,
34054,
198,
220,
220,
220,
11629,
3712,
33,
970,
1303,
19846,
24415,
4235,
198,
220,
220,
220,
279,
3712,
43879,
2414,
1303,
24415,
3124,
28622,
198,
220,
220,
220,
649,
83,
3712,
33,
970,
1303,
19846,
17321,
4235,
198,
220,
220,
220,
285,
3712,
15057,
1303,
649,
1122,
15082,
8467,
5766,
198,
220,
220,
220,
6855,
417,
3712,
33,
970,
1303,
19846,
13314,
417,
7957,
83,
4235,
198,
220,
220,
220,
9403,
3712,
15057,
1303,
13314,
417,
7957,
83,
9403,
1988,
198,
220,
220,
220,
2124,
15,
1303,
13066,
3599,
966,
198,
220,
220,
220,
13066,
3712,
5317,
1303,
13066,
22843,
12384,
6795,
198,
220,
220,
220,
6795,
3712,
5317,
1303,
6795,
286,
2163,
11742,
198,
220,
220,
220,
269,
8899,
3712,
10100,
1303,
545,
12860,
3124,
3975,
198,
220,
220,
220,
6614,
3712,
33,
970,
1303,
10385,
5128,
11898,
284,
2063,
12,
14382,
198,
220,
220,
220,
11898,
3712,
33,
970,
1303,
10385,
5072,
2063,
12,
14382,
284,
11898,
198,
220,
220,
220,
2163,
2896,
500,
7,
36,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1195,
28,
37498,
8937,
17,
7,
89,
36911,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
327,
28,
25,
19510,
9248,
7,
89,
20679,
7,
17,
46582,
4008,
9,
77,
61,
79,
828,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
18872,
224,
28,
46582,
14,
17,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
299,
3712,
46541,
28,
24096,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
399,
3712,
46541,
28,
2327,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
18074,
113,
3712,
15057,
28,
19,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
11629,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
279,
3712,
15057,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
83,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
285,
3712,
15057,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6855,
417,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
9403,
3712,
15057,
28,
15,
13,
15,
10,
15,
13,
15,
320,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
15,
28,
22366,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
13066,
3712,
5317,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6795,
3712,
5317,
28,
16,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
8899,
3712,
10100,
2625,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6614,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
11898,
3712,
33,
970,
28,
9562,
8,
198,
220,
220,
220,
220,
220,
220,
220,
5145,
7,
4906,
1659,
7,
24861,
224,
8,
1279,
25,
15690,
8,
11405,
357,
24861,
224,
796,
25915,
22468,
7,
24861,
224,
828,
24861,
224,
12095,
24861,
224,
11,
24861,
224,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
4129,
7,
24861,
224,
8,
6624,
362,
11405,
357,
24861,
224,
796,
685,
24861,
224,
58,
16,
4357,
24861,
224,
58,
17,
4357,
24861,
224,
58,
16,
4357,
24861,
224,
58,
17,
11907,
8,
198,
220,
220,
220,
220,
220,
220,
220,
5145,
3605,
83,
5633,
357,
69,
796,
2429,
12543,
7,
36,
17414,
25,
89,
11,
25,
66,
36563,
10662,
796,
2429,
12543,
7,
48,
17414,
25,
89,
11,
25,
66,
60,
4008,
1058,
198,
220,
220,
220,
220,
220,
220,
220,
357,
69,
796,
2429,
12543,
7,
3605,
1122,
62,
1470,
1559,
7,
36,
11,
76,
828,
58,
25,
89,
11,
25,
66,
36563,
10662,
796,
2429,
12543,
7,
3109,
1050,
7,
25,
13345,
11,
25,
8937,
11,
36,
828,
58,
25,
89,
11,
25,
66,
60,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
269,
796,
2429,
12543,
7,
34,
17414,
25,
89,
11,
25,
77,
11,
25,
79,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
304,
796,
2099,
1659,
7,
36,
8,
6624,
10903,
5633,
21136,
7,
36,
8,
1058,
412,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
649,
90,
4906,
1659,
7,
69,
828,
4906,
1659,
7,
80,
828,
4906,
1659,
7,
66,
828,
22249,
417,
11,
3605,
83,
11,
14382,
11,
39531,
92,
7,
68,
11,
69,
11,
80,
11,
66,
11,
1102,
1851,
7,
19182,
90,
43879,
2414,
11,
16,
5512,
24861,
224,
828,
52,
5317,
1433,
7,
77,
828,
52,
5317,
23,
7,
45,
828,
22468,
7,
139,
113,
828,
2676,
11,
22468,
7,
79,
828,
3605,
83,
11,
76,
11,
22249,
417,
11,
28826,
11,
87,
15,
11,
42594,
11,
18053,
11,
66,
8899,
11,
14382,
11,
39531,
8,
198,
220,
220,
220,
886,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
12301,
280,
13,
37,
2967,
7248,
7,
3712,
33804,
280,
13,
7469,
500,
8,
198,
198,
7293,
1133,
262,
4600,
33804,
280,
13,
37,
2967,
7248,
63,
900,
1262,
4600,
33804,
280,
13,
7469,
500,
44646,
198,
37811,
198,
7249,
376,
2967,
7248,
90,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
47,
11,
35,
92,
198,
220,
220,
220,
13634,
3712,
7469,
500,
90,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
47,
11,
35,
92,
198,
220,
220,
220,
900,
3712,
46912,
90,
5377,
11141,
90,
43879,
2414,
11709,
198,
220,
220,
220,
11629,
3712,
46912,
90,
52,
5317,
23,
92,
198,
220,
220,
220,
5022,
3712,
46912,
90,
43879,
2414,
92,
198,
220,
220,
220,
2163,
376,
2967,
7248,
90,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
47,
11,
35,
92,
7,
42,
3712,
7469,
500,
90,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
47,
11,
35,
30072,
810,
1391,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
47,
11,
35,
92,
198,
220,
220,
220,
220,
220,
220,
220,
357,
72,
11,
82,
8,
796,
3082,
1133,
7,
42,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
649,
90,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
47,
11,
35,
92,
7,
42,
11,
82,
11,
72,
11,
36654,
2701,
7,
42,
13,
34,
11,
82,
11,
36654,
2701,
7,
22468,
11,
72,
19571,
42,
13,
45,
828,
42,
13,
79,
4008,
198,
220,
220,
220,
886,
198,
437,
628,
220,
37227,
198,
220,
220,
220,
220,
220,
3735,
280,
7,
3712,
33804,
280,
13,
7469,
500,
8,
198,
198,
7293,
1133,
262,
4600,
33804,
280,
13,
37,
2967,
7248,
63,
900,
1262,
4600,
33804,
280,
13,
7469,
500,
44646,
198,
198,
2,
21066,
198,
15506,
63,
16980,
544,
198,
73,
43640,
29,
3735,
280,
7,
42,
8,
198,
15506,
63,
198,
37811,
198,
17359,
280,
7,
42,
3712,
7469,
500,
90,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
47,
11,
35,
30072,
810,
1391,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
47,
11,
35,
92,
796,
376,
2967,
7248,
90,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
47,
11,
35,
92,
7,
42,
8,
198,
198,
37811,
198,
220,
220,
220,
474,
43640,
20797,
7,
3712,
3109,
1050,
26,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
4165,
3975,
11,
357,
89,
11,
269,
8,
4613,
376,
198,
220,
220,
220,
220,
220,
1195,
3712,
3109,
1050,
220,
220,
220,
220,
796,
36147,
8937,
17,
7,
89,
36911,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
6654,
34054,
11,
357,
89,
11,
269,
8,
4613,
1195,
198,
220,
220,
220,
220,
220,
327,
3712,
3109,
1050,
220,
220,
220,
220,
796,
1058,
19510,
9248,
7,
89,
20679,
7,
17,
46582,
4008,
9,
77,
61,
79,
8,
2,
33988,
11,
357,
89,
11,
299,
28,
2676,
1539,
279,
28,
11201,
2014,
4613,
327,
198,
220,
220,
220,
220,
220,
18872,
224,
220,
220,
220,
796,
18074,
222,
14,
17,
11,
1303,
15690,
90,
43879,
2414,
11,
16,
92,
220,
220,
220,
220,
220,
1303,
347,
3733,
11,
685,
87,
7,
64,
828,
87,
7,
65,
828,
88,
7,
64,
828,
88,
7,
65,
15437,
198,
220,
220,
220,
220,
220,
299,
3712,
46541,
220,
796,
26937,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
16021,
10706,
2173,
198,
220,
220,
220,
220,
220,
399,
3712,
46541,
220,
796,
3439,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
3509,
13,
34820,
198,
220,
220,
220,
220,
220,
18074,
113,
3712,
15057,
220,
220,
796,
604,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
34164,
18074,
113,
12,
39184,
34054,
198,
220,
220,
220,
220,
220,
11629,
3712,
33,
970,
220,
796,
3991,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
19846,
24415,
4235,
198,
220,
220,
220,
220,
220,
279,
3712,
15057,
220,
220,
796,
657,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
24415,
3124,
28622,
198,
220,
220,
220,
220,
220,
2124,
15,
220,
220,
220,
220,
220,
220,
220,
220,
220,
796,
2147,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
13066,
3599,
966,
198,
220,
220,
220,
220,
220,
13066,
3712,
5317,
220,
796,
657,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
13066,
22843,
12384,
6795,
198,
220,
220,
220,
220,
220,
6795,
3712,
5317,
220,
796,
352,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
6795,
286,
2163,
11742,
198,
220,
220,
220,
220,
220,
269,
8899,
3712,
10100,
28,
366,
1600,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
545,
12860,
3124,
3975,
198,
220,
220,
220,
220,
220,
6614,
3712,
33,
970,
796,
3991,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
10385,
5128,
11898,
284,
2063,
12,
14382,
198,
220,
220,
220,
220,
220,
11898,
3712,
33,
970,
220,
796,
3991,
8,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
10385,
5072,
2063,
12,
14382,
284,
11898,
198,
198,
63,
7469,
500,
63,
5901,
22300,
34164,
287,
4600,
33804,
280,
63,
198,
198,
2,
1475,
2611,
2374,
198,
15506,
63,
16980,
544,
198,
73,
43640,
29,
474,
43640,
20797,
7203,
89,
61,
17,
12,
15,
13,
3312,
10,
15,
13,
3134,
320,
1600,
24861,
224,
41888,
12,
16,
13,
20,
11,
16,
13,
20,
12095,
16,
11,
16,
4357,
45,
28,
1795,
11,
77,
28,
1314,
486,
11,
66,
8899,
2625,
49,
67,
44802,
4943,
198,
15506,
63,
198,
37811,
198,
8818,
474,
43640,
20797,
7,
36,
26,
198,
220,
220,
220,
220,
220,
220,
220,
1195,
28,
37498,
8937,
17,
7,
89,
36911,
198,
220,
220,
220,
220,
220,
220,
220,
327,
28,
25,
19510,
9248,
7,
89,
20679,
7,
17,
46582,
4008,
9,
77,
61,
79,
828,
198,
220,
220,
220,
220,
220,
220,
220,
18872,
224,
28,
46582,
14,
17,
11,
198,
220,
220,
220,
220,
220,
220,
220,
299,
3712,
46541,
28,
24096,
11,
198,
220,
220,
220,
220,
220,
220,
220,
399,
3712,
46541,
28,
2327,
11,
198,
220,
220,
220,
220,
220,
220,
220,
18074,
113,
3712,
15057,
28,
19,
11,
198,
220,
220,
220,
220,
220,
220,
220,
11629,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
279,
3712,
15057,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
649,
83,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
285,
3712,
15057,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
15,
28,
22366,
11,
198,
220,
220,
220,
220,
220,
220,
220,
13066,
3712,
5317,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
6795,
3712,
5317,
28,
16,
11,
198,
220,
220,
220,
220,
220,
220,
220,
269,
8899,
3712,
10100,
2625,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
6614,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
11898,
3712,
33,
970,
28,
9562,
8,
198,
220,
220,
220,
1441,
2896,
500,
7,
36,
11,
48,
28,
48,
11,
34,
28,
34,
11,
24861,
224,
28,
24861,
224,
11,
77,
28,
77,
11,
45,
28,
45,
11,
139,
113,
28,
139,
113,
11,
2676,
28,
2676,
11,
79,
28,
79,
11,
3605,
83,
28,
3605,
83,
11,
76,
28,
76,
11,
87,
15,
28,
87,
15,
11,
42594,
28,
42594,
11,
18053,
28,
18053,
11,
66,
8899,
28,
66,
8899,
11,
14382,
28,
14382,
11,
39531,
28,
39531,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
6855,
417,
7957,
83,
7,
3712,
3109,
1050,
26,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
4165,
3975,
11,
357,
89,
11,
269,
8,
4613,
376,
198,
220,
220,
220,
220,
220,
1195,
3712,
3109,
1050,
220,
220,
220,
220,
796,
36147,
8937,
17,
7,
89,
36911,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
6654,
34054,
11,
357,
89,
11,
269,
8,
4613,
1195,
198,
220,
220,
220,
220,
220,
327,
3712,
3109,
1050,
220,
220,
220,
220,
796,
36147,
11201,
32590,
8937,
7,
89,
4008,
9,
77,
61,
79,
828,
220,
1303,
33988,
11,
357,
89,
11,
299,
28,
2676,
1539,
279,
28,
11201,
2014,
4613,
327,
198,
220,
220,
220,
220,
220,
18872,
224,
220,
220,
220,
796,
18074,
222,
14,
17,
11,
1303,
15690,
90,
43879,
2414,
11,
16,
92,
220,
220,
220,
220,
220,
1303,
347,
3733,
11,
685,
87,
7,
64,
828,
87,
7,
65,
828,
88,
7,
64,
828,
88,
7,
65,
15437,
198,
220,
220,
220,
220,
220,
299,
3712,
46541,
220,
796,
26937,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
16021,
10706,
2173,
198,
220,
220,
220,
220,
220,
399,
3712,
46541,
220,
796,
3439,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
3509,
13,
34820,
198,
220,
220,
220,
220,
220,
18074,
113,
3712,
15057,
220,
220,
796,
604,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
34164,
18074,
113,
12,
39184,
34054,
198,
220,
220,
220,
220,
220,
11629,
3712,
33,
970,
220,
796,
3991,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
19846,
24415,
4235,
198,
220,
220,
220,
220,
220,
279,
3712,
15057,
220,
220,
796,
657,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
24415,
3124,
28622,
198,
220,
220,
220,
220,
220,
285,
3712,
15057,
220,
220,
796,
657,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
17321,
15082,
8467,
5766,
198,
220,
220,
220,
220,
220,
9403,
3712,
15057,
28,
657,
13,
15,
10,
15,
13,
15,
320,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
13314,
417,
7957,
83,
9403,
1988,
198,
220,
220,
220,
220,
220,
2124,
15,
220,
220,
220,
220,
220,
220,
220,
220,
220,
796,
2147,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
13066,
3599,
966,
198,
220,
220,
220,
220,
220,
13066,
3712,
5317,
220,
796,
657,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
13066,
22843,
12384,
6795,
198,
220,
220,
220,
220,
220,
6795,
3712,
5317,
220,
796,
352,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
6795,
286,
2163,
11742,
198,
220,
220,
220,
220,
220,
269,
8899,
3712,
10100,
28,
366,
1600,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
545,
12860,
3124,
3975,
198,
220,
220,
220,
220,
220,
6614,
3712,
33,
970,
796,
3991,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
10385,
5128,
11898,
284,
2063,
12,
14382,
198,
220,
220,
220,
220,
220,
11898,
3712,
33,
970,
220,
796,
3991,
8,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
10385,
5072,
2063,
12,
14382,
284,
11898,
198,
198,
63,
7469,
500,
63,
13314,
417,
7957,
83,
34164,
287,
4600,
33804,
280,
63,
198,
198,
2,
21066,
198,
15506,
63,
16980,
544,
198,
22249,
417,
7957,
83,
7,
37498,
89,
61,
17,
10,
66,
828,
77,
28,
7410,
11,
45,
28,
1238,
11,
24861,
224,
41888,
12,
16,
13,
6420,
11,
15,
13,
4349,
12095,
16,
13,
2481,
11,
16,
13,
2481,
4357,
66,
8899,
2625,
77,
541,
88,
62,
4443,
1373,
4943,
198,
15506,
63,
198,
37811,
198,
8818,
6855,
417,
7957,
83,
7,
36,
26,
198,
220,
220,
220,
220,
220,
220,
220,
1195,
28,
37498,
8937,
17,
7,
89,
36911,
198,
220,
220,
220,
220,
220,
220,
220,
18872,
224,
28,
46582,
14,
17,
11,
198,
220,
220,
220,
220,
220,
220,
220,
327,
28,
37498,
11201,
32590,
8937,
7,
89,
4008,
9,
77,
61,
79,
828,
198,
220,
220,
220,
220,
220,
220,
220,
299,
3712,
46541,
28,
24096,
11,
198,
220,
220,
220,
220,
220,
220,
220,
399,
3712,
46541,
28,
2327,
11,
198,
220,
220,
220,
220,
220,
220,
220,
18074,
113,
3712,
15057,
28,
19,
11,
198,
220,
220,
220,
220,
220,
220,
220,
11629,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
279,
3712,
15057,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
649,
83,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
285,
3712,
15057,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
9403,
3712,
15057,
28,
15,
13,
15,
10,
15,
13,
15,
320,
11,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
15,
28,
22366,
11,
198,
220,
220,
220,
220,
220,
220,
220,
13066,
3712,
5317,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
6795,
3712,
5317,
28,
16,
11,
198,
220,
220,
220,
220,
220,
220,
220,
269,
8899,
3712,
10100,
2625,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
6614,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
11898,
3712,
33,
970,
28,
9562,
8,
198,
220,
220,
220,
285,
15139,
254,
657,
11405,
357,
3605,
83,
796,
2081,
8,
198,
220,
220,
220,
1441,
2896,
500,
7,
36,
11,
48,
28,
48,
11,
34,
28,
34,
11,
24861,
224,
28,
24861,
224,
11,
77,
28,
77,
11,
45,
28,
45,
11,
139,
113,
28,
139,
113,
11,
2676,
28,
2676,
11,
79,
28,
79,
11,
3605,
83,
28,
3605,
83,
11,
76,
28,
76,
11,
22249,
417,
28,
7942,
11,
28826,
28,
28826,
11,
87,
15,
28,
87,
15,
11,
42594,
28,
42594,
11,
18053,
28,
18053,
11,
66,
8899,
28,
66,
8899,
11,
14382,
28,
14382,
11,
39531,
28,
39531,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
649,
1122,
7,
3712,
3109,
1050,
26,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
4165,
3975,
11,
357,
89,
11,
269,
8,
4613,
376,
198,
220,
220,
220,
220,
220,
327,
3712,
3109,
1050,
220,
220,
220,
220,
796,
1058,
19510,
9248,
7,
89,
20679,
7,
17,
46582,
4008,
9,
77,
61,
79,
8,
2,
33988,
11,
357,
89,
11,
299,
28,
2676,
1539,
279,
28,
11201,
2014,
4613,
327,
198,
220,
220,
220,
220,
220,
18872,
224,
220,
220,
220,
796,
18074,
222,
14,
17,
11,
1303,
15690,
90,
43879,
2414,
11,
16,
92,
220,
220,
220,
220,
220,
1303,
347,
3733,
11,
685,
87,
7,
64,
828,
87,
7,
65,
828,
88,
7,
64,
828,
88,
7,
65,
15437,
198,
220,
220,
220,
220,
220,
299,
3712,
46541,
220,
796,
26937,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
16021,
10706,
2173,
198,
220,
220,
220,
220,
220,
399,
3712,
46541,
220,
796,
3439,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
3509,
13,
34820,
198,
220,
220,
220,
220,
220,
18074,
113,
3712,
15057,
220,
220,
796,
604,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
34164,
18074,
113,
12,
39184,
34054,
198,
220,
220,
220,
220,
220,
11629,
3712,
33,
970,
220,
796,
3991,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
19846,
24415,
4235,
198,
220,
220,
220,
220,
220,
279,
3712,
15057,
220,
220,
796,
657,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
24415,
3124,
28622,
198,
220,
220,
220,
220,
220,
285,
3712,
15057,
220,
220,
796,
657,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
17321,
15082,
8467,
5766,
198,
220,
220,
220,
220,
220,
6855,
417,
3712,
33,
970,
28,
3991,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
19846,
13314,
417,
7957,
83,
4235,
198,
220,
220,
220,
220,
220,
9403,
3712,
15057,
28,
657,
13,
15,
10,
15,
13,
15,
320,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
13314,
417,
7957,
83,
9403,
1988,
198,
220,
220,
220,
220,
220,
2124,
15,
220,
220,
220,
220,
220,
220,
220,
220,
220,
796,
2147,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
13066,
3599,
966,
198,
220,
220,
220,
220,
220,
13066,
3712,
5317,
220,
796,
657,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
13066,
22843,
12384,
6795,
198,
220,
220,
220,
220,
220,
6795,
3712,
5317,
220,
796,
352,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
6795,
286,
2163,
11742,
198,
220,
220,
220,
220,
220,
269,
8899,
3712,
10100,
28,
366,
1600,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
545,
12860,
3124,
3975,
198,
220,
220,
220,
220,
220,
6614,
3712,
33,
970,
796,
3991,
11,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
10385,
5128,
11898,
284,
2063,
12,
14382,
198,
220,
220,
220,
220,
220,
11898,
3712,
33,
970,
220,
796,
3991,
8,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
10385,
5072,
2063,
12,
14382,
284,
11898,
198,
198,
63,
7469,
500,
63,
17321,
34164,
287,
4600,
33804,
280,
63,
198,
198,
2,
21066,
198,
15506,
63,
16980,
544,
198,
73,
43640,
29,
649,
1122,
7203,
89,
61,
18,
12,
16,
1600,
77,
28,
7410,
11,
66,
8899,
2625,
1671,
70,
4943,
198,
15506,
63,
198,
37811,
198,
8818,
649,
1122,
7,
36,
26,
198,
220,
220,
220,
220,
220,
220,
220,
327,
28,
25,
19510,
9248,
7,
89,
20679,
7,
17,
46582,
4008,
9,
77,
61,
79,
828,
198,
220,
220,
220,
220,
220,
220,
220,
18872,
224,
28,
46582,
14,
17,
11,
198,
220,
220,
220,
220,
220,
220,
220,
299,
3712,
46541,
28,
24096,
11,
198,
220,
220,
220,
220,
220,
220,
220,
399,
3712,
46541,
28,
2327,
11,
198,
220,
220,
220,
220,
220,
220,
220,
18074,
113,
3712,
15057,
28,
15,
13,
486,
11,
198,
220,
220,
220,
220,
220,
220,
220,
11629,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
279,
3712,
15057,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
285,
3712,
15057,
28,
16,
11,
198,
220,
220,
220,
220,
220,
220,
220,
6855,
417,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
9403,
3712,
15057,
28,
15,
13,
15,
10,
15,
13,
15,
320,
11,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
15,
28,
22366,
11,
198,
220,
220,
220,
220,
220,
220,
220,
13066,
3712,
5317,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
6795,
3712,
5317,
28,
16,
11,
198,
220,
220,
220,
220,
220,
220,
220,
269,
8899,
3712,
10100,
2625,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
6614,
3712,
33,
970,
28,
9562,
11,
198,
220,
220,
220,
220,
220,
220,
220,
11898,
3712,
33,
970,
28,
9562,
8,
198,
220,
220,
220,
1441,
2896,
500,
7,
36,
11,
34,
28,
34,
11,
24861,
224,
28,
24861,
224,
11,
77,
28,
77,
11,
45,
28,
45,
11,
139,
113,
28,
139,
113,
11,
2676,
28,
2676,
11,
79,
28,
79,
11,
3605,
83,
28,
7942,
11,
76,
28,
76,
11,
22249,
417,
28,
22249,
417,
11,
28826,
28,
28826,
11,
87,
15,
28,
87,
15,
11,
42594,
28,
42594,
11,
18053,
28,
18053,
11,
66,
8899,
28,
66,
8899,
11,
14382,
28,
14382,
11,
39531,
28,
39531,
8,
198,
437,
198,
198,
2,
3440,
3224,
11244,
198,
17256,
7203,
23124,
874,
13,
20362,
15341,
2291,
7203,
42594,
29487,
13,
20362,
4943,
198,
198,
37811,
198,
220,
220,
220,
34164,
7,
3712,
33804,
280,
13,
7469,
500,
11,
7904,
46541,
8,
198,
198,
26410,
262,
4600,
73,
63,
12,
400,
34164,
286,
4600,
33804,
280,
13,
7469,
500,
63,
355,
4689,
49568,
13,
198,
10871,
8840,
24415,
286,
262,
17321,
12,
49,
6570,
1559,
2446,
481,
7800,
257,
517,
8253,
900,
13,
198,
198,
2,
21066,
198,
15506,
63,
16980,
544,
198,
73,
43640,
29,
34164,
7,
3605,
1122,
7203,
89,
61,
18,
12,
16,
12340,
17,
8,
198,
43,
1,
59,
3,
6852,
13812,
7635,
360,
62,
17,
7,
6852,
538,
18217,
261,
8,
796,
26867,
9464,
6852,
90,
89,
6852,
259,
6852,
11018,
11848,
90,
34,
38362,
6852,
9464,
91,
6852,
11,
89,
532,
26867,
31944,
90,
6852,
9464,
7,
89,
532,
26867,
31944,
90,
89,
36796,
18,
92,
532,
352,
18477,
18,
1976,
36796,
17,
11709,
6852,
3506,
8,
36796,
18,
92,
532,
352,
18477,
18,
26867,
9464,
7,
89,
532,
26867,
31944,
90,
89,
36796,
18,
92,
532,
352,
18477,
18,
1976,
36796,
17,
11709,
6852,
3506,
8,
36796,
17,
11709,
532,
26867,
31944,
90,
89,
36796,
18,
92,
532,
352,
18477,
18,
1976,
36796,
17,
11709,
532,
374,
62,
72,
6852,
11,
6852,
3506,
91,
27,
6852,
538,
18217,
261,
11,
6852,
11,
6852,
1640,
439,
374,
62,
72,
7,
6852,
11,
69,
7,
81,
62,
72,
47505,
15,
1267,
6852,
3506,
6852,
32239,
3,
1,
198,
15506,
63,
198,
37811,
198,
12093,
259,
7,
42,
3712,
7469,
500,
11,
73,
8,
796,
509,
13,
3605,
83,
5633,
299,
81,
2617,
7,
42,
13,
36,
11,
42,
13,
76,
11,
73,
8,
1058,
474,
2617,
7,
42,
13,
36,
11,
73,
8,
198,
198,
14382,
7,
89,
3712,
5377,
11141,
8,
796,
357,
17,
89,
13,
260,
29006,
89,
13,
260,
61,
17,
33747,
16,
12,
89,
13,
320,
8,
61,
17,
4008,
10,
320,
9,
7,
16,
12,
89,
13,
260,
61,
17,
12,
89,
13,
320,
61,
17,
20679,
7,
89,
13,
260,
61,
17,
33747,
16,
12,
89,
13,
320,
8,
61,
17,
8,
198,
39531,
7,
89,
3712,
5377,
11141,
8,
796,
357,
17,
89,
13,
260,
29006,
89,
13,
260,
61,
17,
33747,
16,
10,
89,
13,
320,
8,
61,
17,
4008,
10,
320,
9,
7,
89,
13,
260,
61,
17,
10,
89,
13,
320,
61,
17,
12,
16,
20679,
7,
89,
13,
260,
61,
17,
33747,
16,
10,
89,
13,
320,
8,
61,
17,
8,
198,
198,
2,
8160,
2163,
329,
14492,
13066,
286,
257,
1976,
15,
5128,
198,
8818,
13066,
7,
42,
3712,
7469,
500,
90,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
47,
11,
35,
5512,
89,
15,
3712,
5377,
11141,
90,
43879,
2414,
30072,
810,
1391,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
47,
11,
35,
92,
198,
220,
220,
220,
337,
5633,
357,
89,
796,
509,
13,
28826,
8,
1058,
357,
89,
796,
350,
5633,
6614,
7,
89,
15,
8,
1058,
1976,
15,
8,
198,
220,
220,
220,
1976,
77,
796,
657,
87,
405,
198,
220,
220,
220,
981,
357,
45,
5633,
357,
42,
13,
48,
7,
89,
11,
89,
15,
2599,
25,
43879,
2414,
29,
42,
13,
139,
113,
2599,
25,
33,
970,
1058,
357,
42,
13,
48,
7,
89,
11,
89,
15,
2599,
25,
43879,
2414,
27,
42,
13,
139,
113,
8,
2599,
25,
33,
970,
11405,
509,
13,
45,
29,
47347,
198,
220,
220,
220,
220,
220,
220,
220,
1976,
796,
509,
13,
37,
7,
89,
11,
89,
15,
2599,
25,
5377,
11141,
90,
43879,
2414,
92,
198,
220,
220,
220,
220,
220,
220,
220,
1976,
77,
47932,
15,
87,
486,
198,
220,
220,
220,
886,
26,
1303,
437,
198,
220,
220,
220,
1303,
1441,
262,
39279,
4578,
286,
1976,
393,
24415,
954,
198,
220,
220,
220,
1441,
357,
47347,
3712,
52,
5317,
23,
11,
7,
35,
5633,
11898,
7,
89,
8,
1058,
1976,
2599,
25,
5377,
11141,
90,
43879,
2414,
30072,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
3082,
1133,
7,
3712,
33804,
280,
13,
7469,
500,
2599,
25,
38176,
90,
46912,
90,
52,
5317,
23,
5512,
46912,
90,
43879,
2414,
11709,
198,
198,
63,
7293,
1133,
63,
262,
4600,
19182,
63,
329,
4600,
33804,
280,
13,
37,
2967,
7248,
63,
355,
693,
344,
69,
798,
416,
4600,
33804,
280,
13,
7469,
500,
44646,
198,
37811,
198,
8818,
3082,
1133,
7,
42,
3712,
7469,
500,
90,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
35,
92,
2599,
25,
51,
29291,
90,
46912,
90,
52,
5317,
23,
5512,
46912,
90,
5377,
11141,
90,
43879,
2414,
42535,
810,
1391,
9792,
11,
48,
51,
11,
4177,
11,
44,
11,
45,
11,
35,
92,
198,
220,
220,
220,
1303,
7716,
20435,
10706,
198,
220,
220,
220,
509,
2047,
796,
2835,
7,
52,
5317,
1433,
11,
7,
42,
13,
24861,
224,
58,
19,
45297,
42,
13,
24861,
224,
58,
18,
12962,
29006,
42,
13,
24861,
224,
58,
17,
45297,
42,
13,
24861,
224,
58,
16,
12962,
9,
42,
13,
77,
8,
198,
220,
220,
220,
2124,
796,
2837,
7,
42,
13,
24861,
224,
58,
16,
48688,
15,
13,
18005,
11,
11338,
28,
42,
13,
24861,
224,
58,
17,
4357,
13664,
28,
42,
13,
77,
8,
198,
220,
220,
220,
331,
796,
2837,
7,
42,
13,
24861,
224,
58,
19,
4357,
11338,
28,
42,
13,
24861,
224,
58,
18,
4357,
13664,
28,
42,
2047,
8,
198,
220,
220,
220,
1168,
796,
2124,
6,
764,
10,
545,
9,
88,
1303,
4174,
17321,
12,
5574,
2545,
2163,
5002,
12,
3083,
284,
20435,
10706,
198,
220,
220,
220,
357,
6759,
52,
11,
6759,
37,
8,
796,
357,
19182,
90,
52,
5317,
23,
11,
17,
92,
7,
917,
891,
11,
42,
2047,
11,
42,
13,
77,
828,
19182,
90,
5377,
11141,
90,
43879,
2414,
5512,
17,
92,
7,
917,
891,
11,
42,
2047,
11,
42,
13,
77,
4008,
198,
220,
220,
220,
2488,
2435,
2488,
16663,
82,
329,
474,
796,
352,
25,
13664,
7,
88,
1776,
329,
479,
796,
352,
25,
13664,
7,
87,
1776,
198,
220,
220,
220,
220,
220,
220,
220,
357,
6759,
52,
58,
73,
11,
74,
4357,
6759,
37,
58,
73,
11,
74,
12962,
796,
13066,
7,
42,
11,
57,
58,
73,
11,
74,
60,
2599,
25,
51,
29291,
90,
52,
5317,
23,
11,
5377,
11141,
90,
43879,
2414,
11709,
198,
220,
220,
220,
886,
26,
886,
198,
220,
220,
220,
1441,
357,
6759,
52,
11,
6759,
37,
8,
198,
437,
198,
198,
8818,
11593,
15003,
834,
3419,
198,
220,
220,
220,
44872,
7203,
33804,
280,
12326,
29568,
16818,
82,
13,
77,
16663,
82,
28955,
474,
43640,
14390,
19570,
198,
220,
220,
220,
2488,
46115,
9485,
43328,
2625,
67,
26073,
65,
6659,
65,
12,
21,
44705,
12,
4059,
64,
12,
24,
2670,
64,
12,
17,
344,
41544,
44705,
18,
1453,
1,
2291,
7203,
9078,
29487,
13,
20362,
4943,
198,
220,
220,
220,
2488,
46115,
7412,
818,
44798,
282,
2625,
67,
23,
66,
34256,
1795,
12,
1954,
3459,
12,
20,
3559,
65,
12,
23,
66,
5333,
12,
67,
24,
69,
23,
2996,
25191,
24970,
1,
2291,
7203,
4354,
13,
20362,
4943,
198,
220,
220,
220,
2488,
46115,
34371,
3646,
1747,
2625,
65,
3459,
2996,
34159,
12,
10210,
4310,
12,
3553,
2624,
12,
11848,
2327,
12,
5705,
330,
11848,
11785,
23815,
1,
2291,
7203,
403,
24705,
1747,
13,
20362,
4943,
198,
437,
198,
198,
437,
1303,
8265,
198
] | 1.845016 | 6,691 |
using Requests
export
PastebinClient,
PastebinResponse,
PasteKey,
NEVER, TEN_M, HOUR, DAY, WEEK, TWO_WEEKS, MONTH,
PUBLIC, UNLISTED, PRIVATE
@enum Expiration NEVER=1 TEN_M=2 HOUR=3 DAY=4 WEEK=5 TWO_WEEKS=6 MONTH=7
@enum Access PUBLIC=0 UNLISTED=1 PRIVATE=2
immutable PastebinClient
devKey::AbstractString
userKey::Nullable{AbstractString}
PastebinClient(devKey::AbstractString) = new(devKey, Nullable{AbstractString}())
PastebinClient(devKey::AbstractString, userKey::AbstractString) = new(devKey, userKey)
# function to auto generate the user key
function PastebinClient(devKey::AbstractString, username::AbstractString, password::AbstractString)
data = Dict(
"api_dev_key" => devKey,
"api_user_name" => username,
"api_user_password" => password,
)
response = readall(Requests.post("http://pastebin.com/api/api_login.php", data=data))
new(devKey, response)
end
end
immutable PastebinResponse
successful::Bool
response::AbstractString
PastebinResponse(successful::Bool, response::AbstractString) = new(successful, response)
end
immutable PasteKey
key::AbstractString
PasteKey(key::AbstractString) = new(key)
end | [
3500,
9394,
3558,
198,
198,
39344,
198,
220,
220,
220,
29582,
11792,
11,
198,
220,
220,
220,
29582,
31077,
11,
198,
220,
220,
220,
23517,
9218,
11,
198,
220,
220,
220,
33602,
11,
309,
1677,
62,
44,
11,
367,
11698,
11,
24644,
11,
43765,
11,
35288,
62,
54,
6500,
27015,
11,
25000,
4221,
11,
198,
220,
220,
220,
44731,
11,
4725,
45849,
1961,
11,
4810,
3824,
6158,
198,
198,
31,
44709,
1475,
10514,
33602,
28,
16,
309,
1677,
62,
44,
28,
17,
367,
11698,
28,
18,
24644,
28,
19,
43765,
28,
20,
35288,
62,
54,
6500,
27015,
28,
21,
25000,
4221,
28,
22,
198,
31,
44709,
8798,
44731,
28,
15,
4725,
45849,
1961,
28,
16,
4810,
3824,
6158,
28,
17,
198,
198,
8608,
18187,
29582,
11792,
198,
220,
220,
220,
1614,
9218,
3712,
23839,
10100,
198,
220,
220,
220,
2836,
9218,
3712,
35067,
540,
90,
23839,
10100,
92,
628,
220,
220,
220,
29582,
11792,
7,
7959,
9218,
3712,
23839,
10100,
8,
796,
649,
7,
7959,
9218,
11,
35886,
540,
90,
23839,
10100,
92,
28955,
198,
220,
220,
220,
29582,
11792,
7,
7959,
9218,
3712,
23839,
10100,
11,
2836,
9218,
3712,
23839,
10100,
8,
796,
649,
7,
7959,
9218,
11,
2836,
9218,
8,
628,
220,
220,
220,
1303,
2163,
284,
8295,
7716,
262,
2836,
1994,
198,
220,
220,
220,
2163,
29582,
11792,
7,
7959,
9218,
3712,
23839,
10100,
11,
20579,
3712,
23839,
10100,
11,
9206,
3712,
23839,
10100,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1366,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
15042,
62,
7959,
62,
2539,
1,
5218,
1614,
9218,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
15042,
62,
7220,
62,
3672,
1,
5218,
20579,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
15042,
62,
7220,
62,
28712,
1,
5218,
9206,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1267,
628,
220,
220,
220,
220,
220,
220,
220,
2882,
796,
1100,
439,
7,
16844,
3558,
13,
7353,
7203,
4023,
1378,
30119,
23497,
13,
785,
14,
15042,
14,
15042,
62,
38235,
13,
10121,
1600,
1366,
28,
7890,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
649,
7,
7959,
9218,
11,
2882,
8,
198,
220,
220,
220,
886,
198,
198,
437,
198,
198,
8608,
18187,
29582,
31077,
198,
220,
220,
220,
4388,
3712,
33,
970,
198,
220,
220,
220,
2882,
3712,
23839,
10100,
628,
220,
220,
220,
29582,
31077,
7,
17212,
3712,
33,
970,
11,
2882,
3712,
23839,
10100,
8,
796,
649,
7,
17212,
11,
2882,
8,
198,
437,
198,
198,
8608,
18187,
23517,
9218,
198,
220,
220,
220,
1994,
3712,
23839,
10100,
628,
220,
220,
220,
23517,
9218,
7,
2539,
3712,
23839,
10100,
8,
796,
649,
7,
2539,
8,
198,
437
] | 2.688034 | 468 |
using Weave
using Flux
using DataFrames, CSV
using Binning
using Random:shuffle!
using BSON: @save, @load
using Plots
include("./trainModel.jl")
filename = "./evalTemplate.jl"
weave(filename; doctype="md2html", out_path=:pwd)
weave(filename; doctype="github", out_path=:pwd)
| [
3500,
775,
1015,
198,
3500,
1610,
2821,
198,
3500,
6060,
35439,
11,
44189,
198,
3500,
20828,
768,
198,
3500,
14534,
25,
1477,
18137,
0,
198,
3500,
347,
11782,
25,
2488,
21928,
11,
2488,
2220,
198,
3500,
1345,
1747,
198,
17256,
7,
1911,
14,
27432,
17633,
13,
20362,
4943,
198,
198,
34345,
796,
366,
19571,
18206,
30800,
13,
20362,
1,
198,
198,
732,
1015,
7,
34345,
26,
10412,
2981,
2625,
9132,
17,
6494,
1600,
503,
62,
6978,
28,
25,
79,
16993,
8,
198,
732,
1015,
7,
34345,
26,
10412,
2981,
2625,
12567,
1600,
503,
62,
6978,
28,
25,
79,
16993,
8,
198
] | 2.742574 | 101 |
# load packages
using CSV
using Random
"""
set_up(;μ_θ_1::Real = -0.7, τ_θ_1::Real = 1.5, μ_θ_2::Real = 1.5, τ_θ_2::Real = 1.2, μ_θ_3::Real = -0.9, τ_θ_3::Real = 1.5, σ_ϵ::Real = 0.3, dt::Real=0.1, M::Int=5, N::Int=100)
Returns ground-truth parameter values and simulated data from the OU SDEMEM model.
"""
function set_up(;μ_θ_1::Real = -0.7, τ_θ_1::Real = 4,
μ_θ_2::Real = 2.3, τ_θ_2::Real = 10,
μ_θ_3::Real = -0.9, τ_θ_3::Real = 4,
σ_ϵ::Real = 0.3, dt::Real=0.05, M::Int=5, N::Int=100,seed::Int)
# sample random effects
Random.seed!(seed)
ϕ = zeros(M,3)
for i = 1:M
ϕ[i,1] = μ_θ_1 + sqrt(1/τ_θ_1)*randn()
ϕ[i,2] = μ_θ_2 + sqrt(1/τ_θ_2)*randn()
ϕ[i,3] = μ_θ_3 + sqrt(1/τ_θ_3)*randn()
end
# model paramters
η = [μ_θ_1; μ_θ_2; μ_θ_3; τ_θ_1; τ_θ_2; τ_θ_3]
# define priors
μ_0_1 = 0
M_0_1 = 1
α_1 = 2
β_1 = 1
μ_0_2 = 1
M_0_2 = 1
α_2 = 2
β_2 = 1/2
μ_0_3 = 0
M_0_3 = 1
α_3 = 2
β_3 = 1
prior_parameters_η = [μ_0_1 M_0_1 α_1 β_1;μ_0_2 M_0_2 α_2 β_2;μ_0_3 M_0_3 α_3 β_3]
prior_parameters_σ_ϵ = [1; 1/0.4] # Gamma with scale parameter α = 1 and rate parameter β = 1/0.4
# generate data
Random.seed!(seed)
y,x,t_vec = generate_data(N, M, η, ϕ, σ_ϵ,dt)
# return: data, model parameteres, and parameteres for priors
return y,x,t_vec,dt,η,σ_ϵ,ϕ,prior_parameters_η,prior_parameters_σ_ϵ
end
"""
generate_data(N::Int, M::Int, η::Array, ϕ::Array, σ_ϵ::Real,dt::Real)
Generate data from the OU SDEMEM model.
"""
function generate_data(N::Int, M::Int, η::Array, ϕ::Array, σ_ϵ::Real,dt::Real)
x_0 = zeros(M) # pre-allocate matriceis
x = zeros(M,N)
y = zeros(M,N)
t_vec = zeros(N)
for m = 1:M
x[m,1] = x_0[m] # set start value
y[m,1] = x[m,1] + σ_ϵ*randn()
θ_1 = exp(ϕ[m,1]) # set parameters for subject m
θ_2 = exp(ϕ[m,2])
θ_3 = exp(ϕ[m,3])
σ_cond = sqrt((θ_3^2/(2*θ_1))*(1-exp(-2*θ_1*dt))) # set latent process std for subject m
# simulate process for subject m
for t = 2:N
t_vec[t] = t_vec[t-1] + dt
μ_cond = θ_2 + (x[m,t-1]-θ_2)*exp(-θ_1*dt)
x[m,t] = μ_cond + σ_cond*randn()
y[m,t] = x[m,t] + σ_ϵ*randn()
end
end
# return data
return y,x,t_vec
end
| [
2,
3440,
10392,
198,
3500,
44189,
198,
3500,
14534,
198,
198,
37811,
198,
220,
220,
220,
900,
62,
929,
7,
26,
34703,
62,
138,
116,
62,
16,
3712,
15633,
796,
532,
15,
13,
22,
11,
46651,
62,
138,
116,
62,
16,
3712,
15633,
796,
352,
13,
20,
11,
18919,
62,
138,
116,
62,
17,
3712,
15633,
796,
352,
13,
20,
11,
46651,
62,
138,
116,
62,
17,
3712,
15633,
796,
352,
13,
17,
11,
18919,
62,
138,
116,
62,
18,
3712,
15633,
796,
532,
15,
13,
24,
11,
46651,
62,
138,
116,
62,
18,
3712,
15633,
796,
352,
13,
20,
11,
18074,
225,
62,
139,
113,
3712,
15633,
796,
657,
13,
18,
11,
288,
83,
3712,
15633,
28,
15,
13,
16,
11,
337,
3712,
5317,
28,
20,
11,
399,
3712,
5317,
28,
3064,
8,
198,
198,
35561,
2323,
12,
35310,
11507,
3815,
290,
28590,
1366,
422,
262,
47070,
9834,
3620,
3620,
2746,
13,
198,
37811,
198,
8818,
900,
62,
929,
7,
26,
34703,
62,
138,
116,
62,
16,
3712,
15633,
796,
532,
15,
13,
22,
11,
46651,
62,
138,
116,
62,
16,
3712,
15633,
796,
604,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
18919,
62,
138,
116,
62,
17,
3712,
15633,
796,
362,
13,
18,
11,
46651,
62,
138,
116,
62,
17,
3712,
15633,
796,
838,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
18919,
62,
138,
116,
62,
18,
3712,
15633,
796,
532,
15,
13,
24,
11,
46651,
62,
138,
116,
62,
18,
3712,
15633,
796,
604,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
18074,
225,
62,
139,
113,
3712,
15633,
796,
657,
13,
18,
11,
288,
83,
3712,
15633,
28,
15,
13,
2713,
11,
337,
3712,
5317,
28,
20,
11,
399,
3712,
5317,
28,
3064,
11,
28826,
3712,
5317,
8,
628,
198,
220,
220,
220,
1303,
6291,
4738,
3048,
198,
220,
220,
220,
14534,
13,
28826,
0,
7,
28826,
8,
628,
220,
220,
220,
18074,
243,
796,
1976,
27498,
7,
44,
11,
18,
8,
628,
220,
220,
220,
329,
1312,
796,
352,
25,
44,
198,
220,
220,
220,
220,
220,
220,
220,
18074,
243,
58,
72,
11,
16,
60,
796,
18919,
62,
138,
116,
62,
16,
1343,
19862,
17034,
7,
16,
14,
32830,
62,
138,
116,
62,
16,
27493,
25192,
77,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
18074,
243,
58,
72,
11,
17,
60,
796,
18919,
62,
138,
116,
62,
17,
1343,
19862,
17034,
7,
16,
14,
32830,
62,
138,
116,
62,
17,
27493,
25192,
77,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
18074,
243,
58,
72,
11,
18,
60,
796,
18919,
62,
138,
116,
62,
18,
1343,
19862,
17034,
7,
16,
14,
32830,
62,
138,
116,
62,
18,
27493,
25192,
77,
3419,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
2746,
5772,
1010,
198,
220,
220,
220,
7377,
115,
796,
685,
34703,
62,
138,
116,
62,
16,
26,
18919,
62,
138,
116,
62,
17,
26,
18919,
62,
138,
116,
62,
18,
26,
46651,
62,
138,
116,
62,
16,
26,
46651,
62,
138,
116,
62,
17,
26,
46651,
62,
138,
116,
62,
18,
60,
628,
220,
220,
220,
1303,
8160,
1293,
669,
198,
220,
220,
220,
18919,
62,
15,
62,
16,
796,
657,
198,
220,
220,
220,
337,
62,
15,
62,
16,
796,
352,
198,
220,
220,
220,
26367,
62,
16,
796,
362,
198,
220,
220,
220,
27169,
62,
16,
796,
352,
628,
220,
220,
220,
18919,
62,
15,
62,
17,
796,
352,
198,
220,
220,
220,
337,
62,
15,
62,
17,
796,
352,
198,
220,
220,
220,
26367,
62,
17,
796,
362,
198,
220,
220,
220,
27169,
62,
17,
796,
352,
14,
17,
628,
220,
220,
220,
18919,
62,
15,
62,
18,
796,
657,
198,
220,
220,
220,
337,
62,
15,
62,
18,
796,
352,
198,
220,
220,
220,
26367,
62,
18,
796,
362,
198,
220,
220,
220,
27169,
62,
18,
796,
352,
628,
220,
220,
220,
3161,
62,
17143,
7307,
62,
138,
115,
796,
685,
34703,
62,
15,
62,
16,
337,
62,
15,
62,
16,
26367,
62,
16,
27169,
62,
16,
26,
34703,
62,
15,
62,
17,
337,
62,
15,
62,
17,
26367,
62,
17,
27169,
62,
17,
26,
34703,
62,
15,
62,
18,
337,
62,
15,
62,
18,
26367,
62,
18,
27169,
62,
18,
60,
628,
220,
220,
220,
3161,
62,
17143,
7307,
62,
38392,
62,
139,
113,
796,
685,
16,
26,
352,
14,
15,
13,
19,
60,
1303,
43595,
351,
5046,
11507,
26367,
796,
352,
290,
2494,
11507,
27169,
796,
352,
14,
15,
13,
19,
628,
220,
220,
220,
1303,
7716,
1366,
628,
220,
220,
220,
14534,
13,
28826,
0,
7,
28826,
8,
198,
220,
220,
220,
331,
11,
87,
11,
83,
62,
35138,
796,
7716,
62,
7890,
7,
45,
11,
337,
11,
7377,
115,
11,
18074,
243,
11,
18074,
225,
62,
139,
113,
11,
28664,
8,
628,
220,
220,
220,
1303,
1441,
25,
1366,
11,
2746,
5772,
14471,
411,
11,
290,
5772,
14471,
411,
329,
1293,
669,
198,
220,
220,
220,
1441,
331,
11,
87,
11,
83,
62,
35138,
11,
28664,
11,
138,
115,
11,
38392,
62,
139,
113,
11,
139,
243,
11,
3448,
273,
62,
17143,
7307,
62,
138,
115,
11,
3448,
273,
62,
17143,
7307,
62,
38392,
62,
139,
113,
198,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
7716,
62,
7890,
7,
45,
3712,
5317,
11,
337,
3712,
5317,
11,
7377,
115,
3712,
19182,
11,
18074,
243,
3712,
19182,
11,
18074,
225,
62,
139,
113,
3712,
15633,
11,
28664,
3712,
15633,
8,
198,
198,
8645,
378,
1366,
422,
262,
47070,
9834,
3620,
3620,
2746,
13,
198,
37811,
198,
8818,
7716,
62,
7890,
7,
45,
3712,
5317,
11,
337,
3712,
5317,
11,
7377,
115,
3712,
19182,
11,
18074,
243,
3712,
19182,
11,
18074,
225,
62,
139,
113,
3712,
15633,
11,
28664,
3712,
15633,
8,
628,
220,
220,
220,
2124,
62,
15,
796,
1976,
27498,
7,
44,
8,
1303,
662,
12,
439,
13369,
2603,
20970,
271,
198,
220,
220,
220,
2124,
796,
1976,
27498,
7,
44,
11,
45,
8,
198,
220,
220,
220,
331,
796,
1976,
27498,
7,
44,
11,
45,
8,
198,
220,
220,
220,
256,
62,
35138,
796,
1976,
27498,
7,
45,
8,
628,
220,
220,
220,
329,
285,
796,
352,
25,
44,
628,
220,
220,
220,
220,
220,
220,
220,
2124,
58,
76,
11,
16,
60,
796,
2124,
62,
15,
58,
76,
60,
1303,
900,
923,
1988,
198,
220,
220,
220,
220,
220,
220,
220,
331,
58,
76,
11,
16,
60,
796,
2124,
58,
76,
11,
16,
60,
1343,
18074,
225,
62,
139,
113,
9,
25192,
77,
3419,
628,
220,
220,
220,
220,
220,
220,
220,
7377,
116,
62,
16,
796,
1033,
7,
139,
243,
58,
76,
11,
16,
12962,
1303,
900,
10007,
329,
2426,
285,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
116,
62,
17,
796,
1033,
7,
139,
243,
58,
76,
11,
17,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
7377,
116,
62,
18,
796,
1033,
7,
139,
243,
58,
76,
11,
18,
12962,
628,
220,
220,
220,
220,
220,
220,
220,
18074,
225,
62,
17561,
796,
19862,
17034,
19510,
138,
116,
62,
18,
61,
17,
29006,
17,
9,
138,
116,
62,
16,
4008,
9,
7,
16,
12,
11201,
32590,
17,
9,
138,
116,
62,
16,
9,
28664,
22305,
1303,
900,
41270,
1429,
14367,
329,
2426,
285,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
29308,
1429,
329,
2426,
285,
198,
220,
220,
220,
220,
220,
220,
220,
329,
256,
796,
362,
25,
45,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
256,
62,
35138,
58,
83,
60,
796,
256,
62,
35138,
58,
83,
12,
16,
60,
1343,
288,
83,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
18919,
62,
17561,
796,
7377,
116,
62,
17,
1343,
357,
87,
58,
76,
11,
83,
12,
16,
45297,
138,
116,
62,
17,
27493,
11201,
32590,
138,
116,
62,
16,
9,
28664,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
58,
76,
11,
83,
60,
796,
18919,
62,
17561,
1343,
18074,
225,
62,
17561,
9,
25192,
77,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
331,
58,
76,
11,
83,
60,
796,
2124,
58,
76,
11,
83,
60,
1343,
18074,
225,
62,
139,
113,
9,
25192,
77,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
1441,
1366,
198,
220,
220,
220,
1441,
331,
11,
87,
11,
83,
62,
35138,
198,
198,
437,
198
] | 1.657005 | 1,449 |
using Tokenize
# –––––––––––––––
# Some file utils
# –––––––––––––––
function readdir′(dir)
try
readdir(dir)
catch e
String[]
end
end
isdir′(f) = try isdir(f) catch e false end
isfile′(f) = try isfile(f) catch e false end
files(dir) =
@as x dir readdir′(x) map!(f->joinpath(dir, f), x ,x) filter!(isfile′, x)
dirs(dir) =
@as x dir readdir′(x) filter!(f->!startswith(f, "."), x) map!(f->joinpath(dir, f), x, x) filter!(isdir′, x)
jl_files(dir::AbstractString) = @>> dir files filter!(f->endswith(f, ".jl"))
function jl_files(set)
files = Set{String}()
for dir in set, file in jl_files(dir)
push!(files, file)
end
return files
end
# Recursion + Mutable State = Job Security
"""
Takes a start directory and returns a set of nearby directories.
"""
function dirsnearby(dir; descend = 1, ascend = 5, set = Set{String}())
push!(set, dir)
if descend > 0
for down in dirs(dir)
if !(down in set)
push!(set, down)
descend > 1 && dirsnearby(down, descend = descend-1, ascend = 0, set = set)
end
end
end
ascend > 0 && dirname(dir) !== dir && dirsnearby(dirname(dir), descend = descend, ascend = ascend-1, set = set)
return set
end
# ––––––––––––––
# The Good Stuff
# ––––––––––––––
const SCOPE_STARTERS = [Tokens.BEGIN,
Tokens.WHILE,
Tokens.IF,
Tokens.FOR,
Tokens.TRY,
Tokens.FUNCTION,
Tokens.MACRO,
Tokens.LET,
Tokens.TYPE,
Tokens.DO,
Tokens.QUOTE,
Tokens.STRUCT]
const MODULE_STARTERS = [Tokens.MODULE, Tokens.BAREMODULE]
"""
Takes Julia source code and a line number, gives back the string name
of the module at that line.
"""
function codemodule(code, line)
stack = String[]
# count all unterminated block openers, brackets, and parens
openers = [[0,0,0]]
n_openers = 0
n_brackets = 0
n_parens = 0
# index of next modulename token
next_modulename = -1
ts = tokenize(code)
last_token = nothing
for (i, t) in enumerate(ts)
Tokens.startpos(t)[1] > line && break
# Ignore everything in brackets or parnetheses, because any scope started in
# them also needs to be closed in them. That way, we don't need special
# handling for comprehensions and `end`-indexing.
if Tokens.kind(t) == Tokens.LSQUARE
openers[length(stack)+1][2] += 1
elseif openers[length(stack)+1][2] > 0
if Tokens.kind(t) == Tokens.RSQUARE
openers[length(stack)+1][2] -= 1
end
elseif Tokens.kind(t) == Tokens.LPAREN
openers[length(stack)+1][3] += 1
elseif openers[length(stack)+1][3] > 0
if Tokens.kind(t) == Tokens.RPAREN
openers[length(stack)+1][3] -= 1
end
elseif Tokens.exactkind(t) in MODULE_STARTERS && (last_token == nothing || Tokens.kind(last_token) == Tokens.WHITESPACE) # new module
next_modulename = i + 2
elseif i == next_modulename && Tokens.kind(t) == Tokens.IDENTIFIER && Tokens.kind(last_token) == Tokens.WHITESPACE
push!(stack, Tokens.untokenize(t))
push!(openers, [0,0,0])
elseif Tokens.exactkind(t) in SCOPE_STARTERS # new non-module scope
openers[length(stack)+1][1] += 1
elseif Tokens.exactkind(t) == Tokens.END # scope ended
if openers[length(stack)+1][1] == 0
!isempty(stack) && pop!(stack)
length(openers) > 1 && pop!(openers)
else
openers[length(stack)+1][1] -= 1
end
end
last_token = t
end
return join(stack, ".")
end
codemodule(code, pos::Cursor) = codemodule(code, pos.line)
"""
Takes a given Julia source file and another (absolute) path, gives the
line on which the path is included in the file or 0.
"""
function includeline(file::AbstractString, included_file::AbstractString)
# check for erroneous self includes, doesn't detect more complex cycles though
file == included_file && return 0
line = 1
tokens = Tokenize.tokenize(read(file, String))
t, state = iterate(tokens)
while true
if Tokens.kind(t) == Tokens.WHITESPACE
line += count(x -> x == '\n', t.val)
elseif Tokens.kind(t) == Tokens.IDENTIFIER && t.val == "include"
t, state = iterate(tokens, state)
if Tokens.kind(t) == Tokens.LPAREN
t, state = iterate(tokens, state)
if Tokens.kind(t) == Tokens.STRING
if normpath(joinpath(dirname(file), chop(t.val, head=1, tail=1))) == included_file
return line
end
end
end
elseif Tokens.kind(t) == Tokens.ENDMARKER
break
end
t, state = iterate(tokens, state)
end
return 0
end
"""
Takes an absolute path to a file and returns the (file, line) where that
file is included or nothing.
"""
function find_include(path::AbstractString)
for file in @> path dirname dirsnearby jl_files
line = -1
try
line = includeline(file, path)
catch err
return nothing
end
line > 0 && (return file, line)
end
end
"""
Takes an absolute path to a file and returns a string
representing the module it belongs to.
"""
function filemodule_(path::AbstractString)
loc = find_include(path)
if loc != nothing
file, line = loc
mod = codemodule(read(file, String), line)
super = filemodule(file)
if super != "" && mod != ""
return "$super.$mod"
else
return super == "" ? mod : super
end
end
return ""
end
const filemodule = memoize(filemodule_)
# Get all modules
function children(m::Module)
return @>> [moduleusings(m); getmodule.(Ref(m), string.(_names(m, all=true, imported=true)))] filter(x->isa(x, Module) && x ≠ m) unique
end
function allchildren(m::Module, cs = Set{Module}())
for c in children(m)
c in cs || (push!(cs, c); allchildren(c, cs))
end
return cs
end
| [
3500,
29130,
1096,
198,
198,
2,
784,
25608,
25608,
25608,
25608,
25608,
25608,
25608,
198,
2,
2773,
2393,
3384,
4487,
198,
2,
784,
25608,
25608,
25608,
25608,
25608,
25608,
25608,
198,
198,
8818,
1100,
15908,
17478,
7,
15908,
8,
198,
220,
1949,
198,
220,
220,
220,
1100,
15908,
7,
15908,
8,
198,
220,
4929,
304,
198,
220,
220,
220,
10903,
21737,
198,
220,
886,
198,
437,
198,
198,
9409,
343,
17478,
7,
69,
8,
796,
1949,
318,
15908,
7,
69,
8,
4929,
304,
3991,
886,
198,
4468,
576,
17478,
7,
69,
8,
796,
1949,
318,
7753,
7,
69,
8,
4929,
304,
3991,
886,
198,
198,
16624,
7,
15908,
8,
796,
198,
220,
2488,
292,
2124,
26672,
1100,
15908,
17478,
7,
87,
8,
3975,
0,
7,
69,
3784,
22179,
6978,
7,
15908,
11,
277,
828,
2124,
837,
87,
8,
8106,
0,
7,
4468,
576,
17478,
11,
2124,
8,
198,
198,
15908,
82,
7,
15908,
8,
796,
198,
220,
2488,
292,
2124,
26672,
1100,
15908,
17478,
7,
87,
8,
8106,
0,
7,
69,
3784,
0,
9688,
2032,
342,
7,
69,
11,
366,
526,
828,
2124,
8,
3975,
0,
7,
69,
3784,
22179,
6978,
7,
15908,
11,
277,
828,
2124,
11,
2124,
8,
8106,
0,
7,
9409,
343,
17478,
11,
2124,
8,
198,
198,
20362,
62,
16624,
7,
15908,
3712,
23839,
10100,
8,
796,
2488,
4211,
26672,
3696,
8106,
0,
7,
69,
3784,
437,
2032,
342,
7,
69,
11,
27071,
20362,
48774,
198,
198,
8818,
474,
75,
62,
16624,
7,
2617,
8,
198,
220,
3696,
796,
5345,
90,
10100,
92,
3419,
198,
220,
329,
26672,
287,
900,
11,
2393,
287,
474,
75,
62,
16624,
7,
15908,
8,
198,
220,
220,
220,
4574,
0,
7,
16624,
11,
2393,
8,
198,
220,
886,
198,
220,
1441,
3696,
198,
437,
198,
198,
2,
3311,
24197,
1343,
13859,
540,
1812,
796,
15768,
4765,
198,
37811,
198,
51,
1124,
257,
923,
8619,
290,
5860,
257,
900,
286,
6716,
29196,
13,
198,
37811,
198,
8818,
26672,
16184,
451,
1525,
7,
15908,
26,
15350,
796,
352,
11,
34800,
796,
642,
11,
900,
796,
5345,
90,
10100,
92,
28955,
198,
220,
4574,
0,
7,
2617,
11,
26672,
8,
198,
220,
611,
15350,
1875,
657,
198,
220,
220,
220,
329,
866,
287,
288,
17062,
7,
15908,
8,
198,
220,
220,
220,
220,
220,
611,
5145,
7,
2902,
287,
900,
8,
198,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
2617,
11,
866,
8,
198,
220,
220,
220,
220,
220,
220,
220,
15350,
1875,
352,
11405,
26672,
16184,
451,
1525,
7,
2902,
11,
15350,
796,
15350,
12,
16,
11,
34800,
796,
657,
11,
900,
796,
900,
8,
198,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
886,
198,
220,
34800,
1875,
657,
11405,
26672,
3672,
7,
15908,
8,
5145,
855,
26672,
11405,
26672,
16184,
451,
1525,
7,
15908,
3672,
7,
15908,
828,
15350,
796,
15350,
11,
34800,
796,
34800,
12,
16,
11,
900,
796,
900,
8,
628,
220,
1441,
900,
198,
437,
198,
198,
2,
784,
25608,
25608,
25608,
25608,
25608,
25608,
1906,
198,
2,
383,
4599,
27864,
198,
2,
784,
25608,
25608,
25608,
25608,
25608,
25608,
1906,
198,
9979,
6374,
32135,
62,
2257,
7227,
4877,
796,
685,
22906,
13,
33,
43312,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
47365,
13,
12418,
41119,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
47365,
13,
5064,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
47365,
13,
13775,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
47365,
13,
40405,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
47365,
13,
42296,
4177,
2849,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
47365,
13,
44721,
13252,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
47365,
13,
28882,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
47365,
13,
25216,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
47365,
13,
18227,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
47365,
13,
10917,
23051,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
47365,
13,
46126,
60,
198,
198,
9979,
33893,
62,
2257,
7227,
4877,
796,
685,
22906,
13,
33365,
24212,
11,
47365,
13,
33,
1503,
3620,
3727,
24212,
60,
198,
198,
37811,
198,
51,
1124,
22300,
2723,
2438,
290,
257,
1627,
1271,
11,
3607,
736,
262,
4731,
1438,
198,
1659,
262,
8265,
379,
326,
1627,
13,
198,
37811,
198,
8818,
14873,
368,
375,
2261,
7,
8189,
11,
1627,
8,
198,
220,
8931,
796,
10903,
21737,
198,
220,
1303,
954,
477,
555,
23705,
515,
2512,
1280,
364,
11,
28103,
11,
290,
279,
5757,
82,
198,
220,
1280,
364,
796,
16410,
15,
11,
15,
11,
15,
11907,
198,
220,
299,
62,
9654,
364,
796,
657,
198,
220,
299,
62,
1671,
25180,
796,
657,
198,
220,
299,
62,
11730,
82,
796,
657,
198,
220,
1303,
6376,
286,
1306,
953,
377,
12453,
11241,
198,
220,
1306,
62,
4666,
377,
12453,
796,
532,
16,
628,
220,
40379,
796,
11241,
1096,
7,
8189,
8,
628,
220,
938,
62,
30001,
796,
2147,
628,
220,
329,
357,
72,
11,
256,
8,
287,
27056,
378,
7,
912,
8,
198,
220,
220,
220,
47365,
13,
9688,
1930,
7,
83,
38381,
16,
60,
1875,
1627,
11405,
2270,
628,
220,
220,
220,
1303,
41032,
2279,
287,
28103,
393,
279,
1501,
316,
39815,
11,
780,
597,
8354,
2067,
287,
198,
220,
220,
220,
1303,
606,
635,
2476,
284,
307,
4838,
287,
606,
13,
1320,
835,
11,
356,
836,
470,
761,
2041,
198,
220,
220,
220,
1303,
9041,
329,
8569,
507,
290,
4600,
437,
63,
12,
9630,
278,
13,
198,
220,
220,
220,
611,
47365,
13,
11031,
7,
83,
8,
6624,
47365,
13,
6561,
10917,
12203,
198,
220,
220,
220,
220,
220,
1280,
364,
58,
13664,
7,
25558,
47762,
16,
7131,
17,
60,
15853,
352,
198,
220,
220,
220,
2073,
361,
1280,
364,
58,
13664,
7,
25558,
47762,
16,
7131,
17,
60,
1875,
657,
198,
220,
220,
220,
220,
220,
611,
47365,
13,
11031,
7,
83,
8,
6624,
47365,
13,
6998,
10917,
12203,
198,
220,
220,
220,
220,
220,
220,
220,
1280,
364,
58,
13664,
7,
25558,
47762,
16,
7131,
17,
60,
48185,
352,
198,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
2073,
361,
47365,
13,
11031,
7,
83,
8,
6624,
47365,
13,
19930,
1503,
1677,
198,
220,
220,
220,
220,
220,
1280,
364,
58,
13664,
7,
25558,
47762,
16,
7131,
18,
60,
15853,
352,
198,
220,
220,
220,
2073,
361,
1280,
364,
58,
13664,
7,
25558,
47762,
16,
7131,
18,
60,
1875,
657,
198,
220,
220,
220,
220,
220,
611,
47365,
13,
11031,
7,
83,
8,
6624,
47365,
13,
20031,
1503,
1677,
198,
220,
220,
220,
220,
220,
220,
220,
1280,
364,
58,
13664,
7,
25558,
47762,
16,
7131,
18,
60,
48185,
352,
198,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
2073,
361,
47365,
13,
1069,
529,
11031,
7,
83,
8,
287,
33893,
62,
2257,
7227,
4877,
11405,
357,
12957,
62,
30001,
6624,
2147,
8614,
47365,
13,
11031,
7,
12957,
62,
30001,
8,
6624,
47365,
13,
12418,
2043,
1546,
47,
11598,
8,
1303,
649,
8265,
198,
220,
220,
220,
220,
220,
1306,
62,
4666,
377,
12453,
796,
1312,
1343,
362,
198,
220,
220,
220,
2073,
361,
1312,
6624,
1306,
62,
4666,
377,
12453,
11405,
47365,
13,
11031,
7,
83,
8,
6624,
47365,
13,
25256,
5064,
38311,
11405,
47365,
13,
11031,
7,
12957,
62,
30001,
8,
6624,
47365,
13,
12418,
2043,
1546,
47,
11598,
198,
220,
220,
220,
220,
220,
4574,
0,
7,
25558,
11,
47365,
13,
2797,
4233,
1096,
7,
83,
4008,
198,
220,
220,
220,
220,
220,
4574,
0,
7,
9654,
364,
11,
685,
15,
11,
15,
11,
15,
12962,
198,
220,
220,
220,
2073,
361,
47365,
13,
1069,
529,
11031,
7,
83,
8,
287,
6374,
32135,
62,
2257,
7227,
4877,
220,
1303,
649,
1729,
12,
21412,
8354,
198,
220,
220,
220,
220,
220,
1280,
364,
58,
13664,
7,
25558,
47762,
16,
7131,
16,
60,
15853,
352,
198,
220,
220,
220,
2073,
361,
47365,
13,
1069,
529,
11031,
7,
83,
8,
6624,
47365,
13,
10619,
220,
1303,
8354,
4444,
198,
220,
220,
220,
220,
220,
611,
1280,
364,
58,
13664,
7,
25558,
47762,
16,
7131,
16,
60,
6624,
657,
198,
220,
220,
220,
220,
220,
220,
220,
5145,
271,
28920,
7,
25558,
8,
11405,
1461,
0,
7,
25558,
8,
198,
220,
220,
220,
220,
220,
220,
220,
4129,
7,
9654,
364,
8,
1875,
352,
11405,
1461,
0,
7,
9654,
364,
8,
198,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
1280,
364,
58,
13664,
7,
25558,
47762,
16,
7131,
16,
60,
48185,
352,
198,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
938,
62,
30001,
796,
256,
198,
220,
886,
198,
220,
1441,
4654,
7,
25558,
11,
366,
19570,
198,
437,
198,
198,
19815,
368,
375,
2261,
7,
8189,
11,
1426,
3712,
34,
21471,
8,
796,
14873,
368,
375,
2261,
7,
8189,
11,
1426,
13,
1370,
8,
198,
198,
37811,
198,
51,
1124,
257,
1813,
22300,
2723,
2393,
290,
1194,
357,
48546,
8,
3108,
11,
3607,
262,
198,
1370,
319,
543,
262,
3108,
318,
3017,
287,
262,
2393,
393,
657,
13,
198,
37811,
198,
8818,
846,
4470,
7,
7753,
3712,
23839,
10100,
11,
3017,
62,
7753,
3712,
23839,
10100,
8,
198,
220,
1303,
2198,
329,
35366,
2116,
3407,
11,
1595,
470,
4886,
517,
3716,
16006,
996,
198,
220,
2393,
6624,
3017,
62,
7753,
11405,
1441,
657,
628,
220,
1627,
796,
352,
198,
220,
16326,
796,
29130,
1096,
13,
30001,
1096,
7,
961,
7,
7753,
11,
10903,
4008,
628,
220,
256,
11,
1181,
796,
11629,
378,
7,
83,
482,
641,
8,
198,
220,
981,
2081,
198,
220,
220,
220,
611,
47365,
13,
11031,
7,
83,
8,
6624,
47365,
13,
12418,
2043,
1546,
47,
11598,
198,
220,
220,
220,
220,
220,
1627,
15853,
954,
7,
87,
4613,
2124,
6624,
705,
59,
77,
3256,
256,
13,
2100,
8,
198,
220,
220,
220,
2073,
361,
47365,
13,
11031,
7,
83,
8,
6624,
47365,
13,
25256,
5064,
38311,
11405,
256,
13,
2100,
6624,
366,
17256,
1,
198,
220,
220,
220,
220,
220,
256,
11,
1181,
796,
11629,
378,
7,
83,
482,
641,
11,
1181,
8,
198,
220,
220,
220,
220,
220,
611,
47365,
13,
11031,
7,
83,
8,
6624,
47365,
13,
19930,
1503,
1677,
198,
220,
220,
220,
220,
220,
220,
220,
256,
11,
1181,
796,
11629,
378,
7,
83,
482,
641,
11,
1181,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
47365,
13,
11031,
7,
83,
8,
6624,
47365,
13,
18601,
2751,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
2593,
6978,
7,
22179,
6978,
7,
15908,
3672,
7,
7753,
828,
30506,
7,
83,
13,
2100,
11,
1182,
28,
16,
11,
7894,
28,
16,
22305,
6624,
3017,
62,
7753,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1441,
1627,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
2073,
361,
47365,
13,
11031,
7,
83,
8,
6624,
47365,
13,
10619,
44,
14175,
1137,
198,
220,
220,
220,
220,
220,
2270,
198,
220,
220,
220,
886,
198,
220,
220,
220,
256,
11,
1181,
796,
11629,
378,
7,
83,
482,
641,
11,
1181,
8,
198,
220,
886,
198,
220,
1441,
657,
198,
437,
198,
198,
37811,
198,
51,
1124,
281,
4112,
3108,
284,
257,
2393,
290,
5860,
262,
357,
7753,
11,
1627,
8,
810,
326,
198,
7753,
318,
3017,
393,
2147,
13,
198,
37811,
198,
8818,
1064,
62,
17256,
7,
6978,
3712,
23839,
10100,
8,
198,
220,
329,
2393,
287,
2488,
29,
3108,
26672,
3672,
26672,
16184,
451,
1525,
474,
75,
62,
16624,
198,
220,
220,
220,
1627,
796,
532,
16,
198,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
1627,
796,
846,
4470,
7,
7753,
11,
3108,
8,
198,
220,
220,
220,
4929,
11454,
198,
220,
220,
220,
220,
220,
1441,
2147,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1627,
1875,
657,
11405,
357,
7783,
2393,
11,
1627,
8,
198,
220,
886,
198,
437,
198,
198,
37811,
198,
51,
1124,
281,
4112,
3108,
284,
257,
2393,
290,
5860,
257,
4731,
198,
15603,
278,
262,
8265,
340,
14448,
284,
13,
198,
37811,
198,
8818,
2393,
21412,
41052,
6978,
3712,
23839,
10100,
8,
198,
220,
1179,
796,
1064,
62,
17256,
7,
6978,
8,
198,
220,
611,
1179,
14512,
2147,
198,
220,
220,
220,
2393,
11,
1627,
796,
1179,
198,
220,
220,
220,
953,
796,
14873,
368,
375,
2261,
7,
961,
7,
7753,
11,
10903,
828,
1627,
8,
198,
220,
220,
220,
2208,
796,
2393,
21412,
7,
7753,
8,
198,
220,
220,
220,
611,
2208,
14512,
13538,
11405,
953,
14512,
13538,
198,
220,
220,
220,
220,
220,
1441,
17971,
16668,
48082,
4666,
1,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
1441,
2208,
6624,
13538,
5633,
953,
1058,
2208,
198,
220,
220,
220,
886,
198,
220,
886,
198,
220,
1441,
13538,
198,
437,
198,
198,
9979,
2393,
21412,
796,
16155,
1096,
7,
7753,
21412,
62,
8,
198,
198,
2,
3497,
477,
13103,
198,
8818,
1751,
7,
76,
3712,
26796,
8,
198,
220,
1441,
2488,
4211,
685,
21412,
385,
654,
7,
76,
1776,
651,
21412,
12195,
8134,
7,
76,
828,
4731,
12195,
62,
14933,
7,
76,
11,
477,
28,
7942,
11,
17392,
28,
7942,
4008,
15437,
8106,
7,
87,
3784,
9160,
7,
87,
11,
19937,
8,
11405,
2124,
15139,
254,
285,
8,
3748,
198,
437,
198,
198,
8818,
477,
17197,
7,
76,
3712,
26796,
11,
50115,
796,
5345,
90,
26796,
92,
28955,
198,
220,
329,
269,
287,
1751,
7,
76,
8,
198,
220,
220,
220,
269,
287,
50115,
8614,
357,
14689,
0,
7,
6359,
11,
269,
1776,
477,
17197,
7,
66,
11,
50115,
4008,
198,
220,
886,
198,
220,
1441,
50115,
198,
437,
198
] | 2.376006 | 2,484 |
# This file is auto-generated by AWSMetadata.jl
using AWS
using AWS.AWSServices: fis
using AWS.Compat
using AWS.UUIDs
"""
create_experiment_template(actions, client_token, description, role_arn, stop_conditions)
create_experiment_template(actions, client_token, description, role_arn, stop_conditions, params::Dict{String,<:Any})
Creates an experiment template. To create a template, specify the following information:
Targets: A target can be a specific resource in your AWS environment, or one or more
resources that match criteria that you specify, for example, resources that have specific
tags. Actions: The actions to carry out on the target. You can specify multiple actions,
the duration of each action, and when to start each action during an experiment. Stop
conditions: If a stop condition is triggered while an experiment is running, the experiment
is automatically stopped. You can define a stop condition as a CloudWatch alarm. For more
information, see the AWS Fault Injection Simulator User Guide.
# Arguments
- `actions`: The actions for the experiment.
- `client_token`: Unique, case-sensitive identifier that you provide to ensure the
idempotency of the request.
- `description`: A description for the experiment template. Can contain up to 64 letters
(A-Z and a-z).
- `role_arn`: The Amazon Resource Name (ARN) of an IAM role that grants the AWS FIS service
permission to perform service actions on your behalf.
- `stop_conditions`: The stop conditions.
# Optional Parameters
Optional parameters can be passed as a `params::Dict{String,<:Any}`. Valid keys are:
- `"tags"`: The tags to apply to the experiment template.
- `"targets"`: The targets for the experiment.
"""
function create_experiment_template(
actions,
clientToken,
description,
roleArn,
stopConditions;
aws_config::AbstractAWSConfig=global_aws_config(),
)
return fis(
"POST",
"/experimentTemplates",
Dict{String,Any}(
"actions" => actions,
"clientToken" => clientToken,
"description" => description,
"roleArn" => roleArn,
"stopConditions" => stopConditions,
);
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
function create_experiment_template(
actions,
clientToken,
description,
roleArn,
stopConditions,
params::AbstractDict{String};
aws_config::AbstractAWSConfig=global_aws_config(),
)
return fis(
"POST",
"/experimentTemplates",
Dict{String,Any}(
mergewith(
_merge,
Dict{String,Any}(
"actions" => actions,
"clientToken" => clientToken,
"description" => description,
"roleArn" => roleArn,
"stopConditions" => stopConditions,
),
params,
),
);
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
"""
delete_experiment_template(id)
delete_experiment_template(id, params::Dict{String,<:Any})
Deletes the specified experiment template.
# Arguments
- `id`: The ID of the experiment template.
"""
function delete_experiment_template(id; aws_config::AbstractAWSConfig=global_aws_config())
return fis(
"DELETE",
"/experimentTemplates/$(id)";
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
function delete_experiment_template(
id, params::AbstractDict{String}; aws_config::AbstractAWSConfig=global_aws_config()
)
return fis(
"DELETE",
"/experimentTemplates/$(id)",
params;
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
"""
get_action(id)
get_action(id, params::Dict{String,<:Any})
Gets information about the specified AWS FIS action.
# Arguments
- `id`: The ID of the action.
"""
function get_action(id; aws_config::AbstractAWSConfig=global_aws_config())
return fis(
"GET", "/actions/$(id)"; aws_config=aws_config, feature_set=SERVICE_FEATURE_SET
)
end
function get_action(
id, params::AbstractDict{String}; aws_config::AbstractAWSConfig=global_aws_config()
)
return fis(
"GET",
"/actions/$(id)",
params;
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
"""
get_experiment(id)
get_experiment(id, params::Dict{String,<:Any})
Gets information about the specified experiment.
# Arguments
- `id`: The ID of the experiment.
"""
function get_experiment(id; aws_config::AbstractAWSConfig=global_aws_config())
return fis(
"GET", "/experiments/$(id)"; aws_config=aws_config, feature_set=SERVICE_FEATURE_SET
)
end
function get_experiment(
id, params::AbstractDict{String}; aws_config::AbstractAWSConfig=global_aws_config()
)
return fis(
"GET",
"/experiments/$(id)",
params;
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
"""
get_experiment_template(id)
get_experiment_template(id, params::Dict{String,<:Any})
Gets information about the specified experiment template.
# Arguments
- `id`: The ID of the experiment template.
"""
function get_experiment_template(id; aws_config::AbstractAWSConfig=global_aws_config())
return fis(
"GET",
"/experimentTemplates/$(id)";
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
function get_experiment_template(
id, params::AbstractDict{String}; aws_config::AbstractAWSConfig=global_aws_config()
)
return fis(
"GET",
"/experimentTemplates/$(id)",
params;
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
"""
list_actions()
list_actions(params::Dict{String,<:Any})
Lists the available AWS FIS actions.
# Optional Parameters
Optional parameters can be passed as a `params::Dict{String,<:Any}`. Valid keys are:
- `"maxResults"`: The maximum number of results to return with a single call. To retrieve
the remaining results, make another call with the returned nextToken value.
- `"nextToken"`: The token for the next page of results.
"""
function list_actions(; aws_config::AbstractAWSConfig=global_aws_config())
return fis("GET", "/actions"; aws_config=aws_config, feature_set=SERVICE_FEATURE_SET)
end
function list_actions(
params::AbstractDict{String}; aws_config::AbstractAWSConfig=global_aws_config()
)
return fis(
"GET", "/actions", params; aws_config=aws_config, feature_set=SERVICE_FEATURE_SET
)
end
"""
list_experiment_templates()
list_experiment_templates(params::Dict{String,<:Any})
Lists your experiment templates.
# Optional Parameters
Optional parameters can be passed as a `params::Dict{String,<:Any}`. Valid keys are:
- `"maxResults"`: The maximum number of results to return with a single call. To retrieve
the remaining results, make another call with the returned nextToken value.
- `"nextToken"`: The token for the next page of results.
"""
function list_experiment_templates(; aws_config::AbstractAWSConfig=global_aws_config())
return fis(
"GET",
"/experimentTemplates";
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
function list_experiment_templates(
params::AbstractDict{String}; aws_config::AbstractAWSConfig=global_aws_config()
)
return fis(
"GET",
"/experimentTemplates",
params;
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
"""
list_experiments()
list_experiments(params::Dict{String,<:Any})
Lists your experiments.
# Optional Parameters
Optional parameters can be passed as a `params::Dict{String,<:Any}`. Valid keys are:
- `"maxResults"`: The maximum number of results to return with a single call. To retrieve
the remaining results, make another call with the returned nextToken value.
- `"nextToken"`: The token for the next page of results.
"""
function list_experiments(; aws_config::AbstractAWSConfig=global_aws_config())
return fis(
"GET", "/experiments"; aws_config=aws_config, feature_set=SERVICE_FEATURE_SET
)
end
function list_experiments(
params::AbstractDict{String}; aws_config::AbstractAWSConfig=global_aws_config()
)
return fis(
"GET",
"/experiments",
params;
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
"""
list_tags_for_resource(resource_arn)
list_tags_for_resource(resource_arn, params::Dict{String,<:Any})
Lists the tags for the specified resource.
# Arguments
- `resource_arn`: The Amazon Resource Name (ARN) of the resource.
"""
function list_tags_for_resource(
resourceArn; aws_config::AbstractAWSConfig=global_aws_config()
)
return fis(
"GET",
"/tags/$(resourceArn)";
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
function list_tags_for_resource(
resourceArn,
params::AbstractDict{String};
aws_config::AbstractAWSConfig=global_aws_config(),
)
return fis(
"GET",
"/tags/$(resourceArn)",
params;
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
"""
start_experiment(client_token, experiment_template_id)
start_experiment(client_token, experiment_template_id, params::Dict{String,<:Any})
Starts running an experiment from the specified experiment template.
# Arguments
- `client_token`: Unique, case-sensitive identifier that you provide to ensure the
idempotency of the request.
- `experiment_template_id`: The ID of the experiment template.
# Optional Parameters
Optional parameters can be passed as a `params::Dict{String,<:Any}`. Valid keys are:
- `"tags"`: The tags to apply to the experiment.
"""
function start_experiment(
clientToken, experimentTemplateId; aws_config::AbstractAWSConfig=global_aws_config()
)
return fis(
"POST",
"/experiments",
Dict{String,Any}(
"clientToken" => clientToken, "experimentTemplateId" => experimentTemplateId
);
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
function start_experiment(
clientToken,
experimentTemplateId,
params::AbstractDict{String};
aws_config::AbstractAWSConfig=global_aws_config(),
)
return fis(
"POST",
"/experiments",
Dict{String,Any}(
mergewith(
_merge,
Dict{String,Any}(
"clientToken" => clientToken,
"experimentTemplateId" => experimentTemplateId,
),
params,
),
);
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
"""
stop_experiment(id)
stop_experiment(id, params::Dict{String,<:Any})
Stops the specified experiment.
# Arguments
- `id`: The ID of the experiment.
"""
function stop_experiment(id; aws_config::AbstractAWSConfig=global_aws_config())
return fis(
"DELETE",
"/experiments/$(id)";
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
function stop_experiment(
id, params::AbstractDict{String}; aws_config::AbstractAWSConfig=global_aws_config()
)
return fis(
"DELETE",
"/experiments/$(id)",
params;
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
"""
tag_resource(resource_arn, tags)
tag_resource(resource_arn, tags, params::Dict{String,<:Any})
Applies the specified tags to the specified resource.
# Arguments
- `resource_arn`: The Amazon Resource Name (ARN) of the resource.
- `tags`: The tags for the resource.
"""
function tag_resource(resourceArn, tags; aws_config::AbstractAWSConfig=global_aws_config())
return fis(
"POST",
"/tags/$(resourceArn)",
Dict{String,Any}("tags" => tags);
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
function tag_resource(
resourceArn,
tags,
params::AbstractDict{String};
aws_config::AbstractAWSConfig=global_aws_config(),
)
return fis(
"POST",
"/tags/$(resourceArn)",
Dict{String,Any}(mergewith(_merge, Dict{String,Any}("tags" => tags), params));
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
"""
untag_resource(resource_arn)
untag_resource(resource_arn, params::Dict{String,<:Any})
Removes the specified tags from the specified resource.
# Arguments
- `resource_arn`: The Amazon Resource Name (ARN) of the resource.
# Optional Parameters
Optional parameters can be passed as a `params::Dict{String,<:Any}`. Valid keys are:
- `"tagKeys"`: The tag keys to remove.
"""
function untag_resource(resourceArn; aws_config::AbstractAWSConfig=global_aws_config())
return fis(
"DELETE",
"/tags/$(resourceArn)";
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
function untag_resource(
resourceArn,
params::AbstractDict{String};
aws_config::AbstractAWSConfig=global_aws_config(),
)
return fis(
"DELETE",
"/tags/$(resourceArn)",
params;
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
"""
update_experiment_template(id)
update_experiment_template(id, params::Dict{String,<:Any})
Updates the specified experiment template.
# Arguments
- `id`: The ID of the experiment template.
# Optional Parameters
Optional parameters can be passed as a `params::Dict{String,<:Any}`. Valid keys are:
- `"actions"`: The actions for the experiment.
- `"description"`: A description for the template.
- `"roleArn"`: The Amazon Resource Name (ARN) of an IAM role that grants the AWS FIS
service permission to perform service actions on your behalf.
- `"stopConditions"`: The stop conditions for the experiment.
- `"targets"`: The targets for the experiment.
"""
function update_experiment_template(id; aws_config::AbstractAWSConfig=global_aws_config())
return fis(
"PATCH",
"/experimentTemplates/$(id)";
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
function update_experiment_template(
id, params::AbstractDict{String}; aws_config::AbstractAWSConfig=global_aws_config()
)
return fis(
"PATCH",
"/experimentTemplates/$(id)",
params;
aws_config=aws_config,
feature_set=SERVICE_FEATURE_SET,
)
end
| [
2,
770,
2393,
318,
8295,
12,
27568,
416,
30865,
9171,
14706,
13,
20362,
198,
3500,
30865,
198,
3500,
30865,
13,
12298,
5432,
712,
1063,
25,
277,
271,
198,
3500,
30865,
13,
40073,
198,
3500,
30865,
13,
52,
27586,
82,
198,
198,
37811,
198,
220,
220,
220,
2251,
62,
23100,
3681,
62,
28243,
7,
4658,
11,
5456,
62,
30001,
11,
6764,
11,
2597,
62,
1501,
11,
2245,
62,
17561,
1756,
8,
198,
220,
220,
220,
2251,
62,
23100,
3681,
62,
28243,
7,
4658,
11,
5456,
62,
30001,
11,
6764,
11,
2597,
62,
1501,
11,
2245,
62,
17561,
1756,
11,
42287,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
16719,
274,
281,
6306,
11055,
13,
220,
1675,
2251,
257,
11055,
11,
11986,
262,
1708,
1321,
25,
198,
220,
31089,
1039,
25,
317,
2496,
460,
307,
257,
2176,
8271,
287,
534,
30865,
2858,
11,
393,
530,
393,
517,
198,
37540,
326,
2872,
9987,
326,
345,
11986,
11,
329,
1672,
11,
4133,
326,
423,
2176,
198,
31499,
13,
220,
220,
220,
24439,
25,
383,
4028,
284,
3283,
503,
319,
262,
2496,
13,
921,
460,
11986,
3294,
4028,
11,
198,
1169,
9478,
286,
1123,
2223,
11,
290,
618,
284,
923,
1123,
2223,
1141,
281,
6306,
13,
220,
220,
220,
13707,
198,
17561,
1756,
25,
1002,
257,
2245,
4006,
318,
13973,
981,
281,
6306,
318,
2491,
11,
262,
6306,
198,
271,
6338,
5025,
13,
921,
460,
8160,
257,
2245,
4006,
355,
257,
10130,
10723,
10436,
13,
220,
220,
1114,
517,
198,
17018,
11,
766,
262,
30865,
40050,
554,
29192,
13942,
11787,
10005,
13,
198,
198,
2,
20559,
2886,
198,
12,
4600,
4658,
63,
25,
383,
4028,
329,
262,
6306,
13,
198,
12,
4600,
16366,
62,
30001,
63,
25,
30015,
11,
1339,
12,
30176,
27421,
326,
345,
2148,
284,
4155,
262,
198,
220,
4686,
368,
13059,
1387,
286,
262,
2581,
13,
198,
12,
4600,
11213,
63,
25,
317,
6764,
329,
262,
6306,
11055,
13,
1680,
3994,
510,
284,
5598,
7475,
198,
220,
357,
32,
12,
57,
290,
257,
12,
89,
737,
198,
12,
4600,
18090,
62,
1501,
63,
25,
383,
6186,
20857,
6530,
357,
1503,
45,
8,
286,
281,
314,
2390,
2597,
326,
11455,
262,
30865,
376,
1797,
2139,
198,
220,
7170,
284,
1620,
2139,
4028,
319,
534,
8378,
13,
198,
12,
4600,
11338,
62,
17561,
1756,
63,
25,
383,
2245,
3403,
13,
198,
198,
2,
32233,
40117,
198,
30719,
10007,
460,
307,
3804,
355,
257,
4600,
37266,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
92,
44646,
48951,
8251,
389,
25,
198,
12,
4600,
1,
31499,
1,
63,
25,
383,
15940,
284,
4174,
284,
262,
6306,
11055,
13,
198,
12,
4600,
1,
83,
853,
1039,
1,
63,
25,
383,
6670,
329,
262,
6306,
13,
198,
37811,
198,
8818,
2251,
62,
23100,
3681,
62,
28243,
7,
198,
220,
220,
220,
4028,
11,
198,
220,
220,
220,
5456,
30642,
11,
198,
220,
220,
220,
6764,
11,
198,
220,
220,
220,
2597,
3163,
77,
11,
198,
220,
220,
220,
2245,
25559,
1756,
26,
198,
220,
220,
220,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
22784,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
32782,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
3681,
12966,
17041,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
360,
713,
90,
10100,
11,
7149,
92,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
4658,
1,
5218,
4028,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
16366,
30642,
1,
5218,
5456,
30642,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
11213,
1,
5218,
6764,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
18090,
3163,
77,
1,
5218,
2597,
3163,
77,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
11338,
25559,
1756,
1,
5218,
2245,
25559,
1756,
11,
198,
220,
220,
220,
220,
220,
220,
220,
5619,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
2251,
62,
23100,
3681,
62,
28243,
7,
198,
220,
220,
220,
4028,
11,
198,
220,
220,
220,
5456,
30642,
11,
198,
220,
220,
220,
6764,
11,
198,
220,
220,
220,
2597,
3163,
77,
11,
198,
220,
220,
220,
2245,
25559,
1756,
11,
198,
220,
220,
220,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
198,
220,
220,
220,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
22784,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
32782,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
3681,
12966,
17041,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
360,
713,
90,
10100,
11,
7149,
92,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4017,
39909,
342,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4808,
647,
469,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
360,
713,
90,
10100,
11,
7149,
92,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
4658,
1,
5218,
4028,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
16366,
30642,
1,
5218,
5456,
30642,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
11213,
1,
5218,
6764,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
18090,
3163,
77,
1,
5218,
2597,
3163,
77,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
11338,
25559,
1756,
1,
5218,
2245,
25559,
1756,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
10612,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
42287,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
10612,
198,
220,
220,
220,
220,
220,
220,
220,
5619,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
12233,
62,
23100,
3681,
62,
28243,
7,
312,
8,
198,
220,
220,
220,
12233,
62,
23100,
3681,
62,
28243,
7,
312,
11,
42287,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
5005,
40676,
262,
7368,
6306,
11055,
13,
198,
198,
2,
20559,
2886,
198,
12,
4600,
312,
63,
25,
383,
4522,
286,
262,
6306,
11055,
13,
198,
198,
37811,
198,
8818,
12233,
62,
23100,
3681,
62,
28243,
7,
312,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
28955,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
7206,
2538,
9328,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
3681,
12966,
17041,
32624,
7,
312,
8,
8172,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
12233,
62,
23100,
3681,
62,
28243,
7,
198,
220,
220,
220,
4686,
11,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
3419,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
7206,
2538,
9328,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
3681,
12966,
17041,
32624,
7,
312,
42501,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
26,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
651,
62,
2673,
7,
312,
8,
198,
220,
220,
220,
651,
62,
2673,
7,
312,
11,
42287,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
38,
1039,
1321,
546,
262,
7368,
30865,
376,
1797,
2223,
13,
198,
198,
2,
20559,
2886,
198,
12,
4600,
312,
63,
25,
383,
4522,
286,
262,
2223,
13,
198,
198,
37811,
198,
8818,
651,
62,
2673,
7,
312,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
28955,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
12813,
4658,
32624,
7,
312,
8,
8172,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
651,
62,
2673,
7,
198,
220,
220,
220,
4686,
11,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
3419,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
4658,
32624,
7,
312,
42501,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
26,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
651,
62,
23100,
3681,
7,
312,
8,
198,
220,
220,
220,
651,
62,
23100,
3681,
7,
312,
11,
42287,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
38,
1039,
1321,
546,
262,
7368,
6306,
13,
198,
198,
2,
20559,
2886,
198,
12,
4600,
312,
63,
25,
383,
4522,
286,
262,
6306,
13,
198,
198,
37811,
198,
8818,
651,
62,
23100,
3681,
7,
312,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
28955,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
12813,
23100,
6800,
32624,
7,
312,
8,
8172,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
651,
62,
23100,
3681,
7,
198,
220,
220,
220,
4686,
11,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
3419,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
6800,
32624,
7,
312,
42501,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
26,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
651,
62,
23100,
3681,
62,
28243,
7,
312,
8,
198,
220,
220,
220,
651,
62,
23100,
3681,
62,
28243,
7,
312,
11,
42287,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
38,
1039,
1321,
546,
262,
7368,
6306,
11055,
13,
198,
198,
2,
20559,
2886,
198,
12,
4600,
312,
63,
25,
383,
4522,
286,
262,
6306,
11055,
13,
198,
198,
37811,
198,
8818,
651,
62,
23100,
3681,
62,
28243,
7,
312,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
28955,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
3681,
12966,
17041,
32624,
7,
312,
8,
8172,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
651,
62,
23100,
3681,
62,
28243,
7,
198,
220,
220,
220,
4686,
11,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
3419,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
3681,
12966,
17041,
32624,
7,
312,
42501,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
26,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
1351,
62,
4658,
3419,
198,
220,
220,
220,
1351,
62,
4658,
7,
37266,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
43,
1023,
262,
1695,
30865,
376,
1797,
4028,
13,
198,
198,
2,
32233,
40117,
198,
30719,
10007,
460,
307,
3804,
355,
257,
4600,
37266,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
92,
44646,
48951,
8251,
389,
25,
198,
12,
4600,
1,
9806,
25468,
1,
63,
25,
383,
5415,
1271,
286,
2482,
284,
1441,
351,
257,
2060,
869,
13,
1675,
19818,
198,
220,
262,
5637,
2482,
11,
787,
1194,
869,
351,
262,
4504,
1306,
30642,
1988,
13,
198,
12,
4600,
1,
19545,
30642,
1,
63,
25,
383,
11241,
329,
262,
1306,
2443,
286,
2482,
13,
198,
37811,
198,
8818,
1351,
62,
4658,
7,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
28955,
198,
220,
220,
220,
1441,
277,
271,
7203,
18851,
1600,
12813,
4658,
8172,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
8,
198,
437,
198,
8818,
1351,
62,
4658,
7,
198,
220,
220,
220,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
3419,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
12813,
4658,
1600,
42287,
26,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
1351,
62,
23100,
3681,
62,
11498,
17041,
3419,
198,
220,
220,
220,
1351,
62,
23100,
3681,
62,
11498,
17041,
7,
37266,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
43,
1023,
534,
6306,
24019,
13,
198,
198,
2,
32233,
40117,
198,
30719,
10007,
460,
307,
3804,
355,
257,
4600,
37266,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
92,
44646,
48951,
8251,
389,
25,
198,
12,
4600,
1,
9806,
25468,
1,
63,
25,
383,
5415,
1271,
286,
2482,
284,
1441,
351,
257,
2060,
869,
13,
1675,
19818,
198,
220,
262,
5637,
2482,
11,
787,
1194,
869,
351,
262,
4504,
1306,
30642,
1988,
13,
198,
12,
4600,
1,
19545,
30642,
1,
63,
25,
383,
11241,
329,
262,
1306,
2443,
286,
2482,
13,
198,
37811,
198,
8818,
1351,
62,
23100,
3681,
62,
11498,
17041,
7,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
28955,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
3681,
12966,
17041,
8172,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
1351,
62,
23100,
3681,
62,
11498,
17041,
7,
198,
220,
220,
220,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
3419,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
3681,
12966,
17041,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
26,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
1351,
62,
23100,
6800,
3419,
198,
220,
220,
220,
1351,
62,
23100,
6800,
7,
37266,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
43,
1023,
534,
10256,
13,
198,
198,
2,
32233,
40117,
198,
30719,
10007,
460,
307,
3804,
355,
257,
4600,
37266,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
92,
44646,
48951,
8251,
389,
25,
198,
12,
4600,
1,
9806,
25468,
1,
63,
25,
383,
5415,
1271,
286,
2482,
284,
1441,
351,
257,
2060,
869,
13,
1675,
19818,
198,
220,
262,
5637,
2482,
11,
787,
1194,
869,
351,
262,
4504,
1306,
30642,
1988,
13,
198,
12,
4600,
1,
19545,
30642,
1,
63,
25,
383,
11241,
329,
262,
1306,
2443,
286,
2482,
13,
198,
37811,
198,
8818,
1351,
62,
23100,
6800,
7,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
28955,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
12813,
23100,
6800,
8172,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
1351,
62,
23100,
6800,
7,
198,
220,
220,
220,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
3419,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
6800,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
26,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
1351,
62,
31499,
62,
1640,
62,
31092,
7,
31092,
62,
1501,
8,
198,
220,
220,
220,
1351,
62,
31499,
62,
1640,
62,
31092,
7,
31092,
62,
1501,
11,
42287,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
43,
1023,
262,
15940,
329,
262,
7368,
8271,
13,
198,
198,
2,
20559,
2886,
198,
12,
4600,
31092,
62,
1501,
63,
25,
383,
6186,
20857,
6530,
357,
1503,
45,
8,
286,
262,
8271,
13,
198,
198,
37811,
198,
8818,
1351,
62,
31499,
62,
1640,
62,
31092,
7,
198,
220,
220,
220,
8271,
3163,
77,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
3419,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
31499,
32624,
7,
31092,
3163,
77,
8,
8172,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
1351,
62,
31499,
62,
1640,
62,
31092,
7,
198,
220,
220,
220,
8271,
3163,
77,
11,
198,
220,
220,
220,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
198,
220,
220,
220,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
22784,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
18851,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
31499,
32624,
7,
31092,
3163,
77,
42501,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
26,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
923,
62,
23100,
3681,
7,
16366,
62,
30001,
11,
6306,
62,
28243,
62,
312,
8,
198,
220,
220,
220,
923,
62,
23100,
3681,
7,
16366,
62,
30001,
11,
6306,
62,
28243,
62,
312,
11,
42287,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
1273,
5889,
2491,
281,
6306,
422,
262,
7368,
6306,
11055,
13,
198,
198,
2,
20559,
2886,
198,
12,
4600,
16366,
62,
30001,
63,
25,
30015,
11,
1339,
12,
30176,
27421,
326,
345,
2148,
284,
4155,
262,
198,
220,
4686,
368,
13059,
1387,
286,
262,
2581,
13,
198,
12,
4600,
23100,
3681,
62,
28243,
62,
312,
63,
25,
383,
4522,
286,
262,
6306,
11055,
13,
198,
198,
2,
32233,
40117,
198,
30719,
10007,
460,
307,
3804,
355,
257,
4600,
37266,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
92,
44646,
48951,
8251,
389,
25,
198,
12,
4600,
1,
31499,
1,
63,
25,
383,
15940,
284,
4174,
284,
262,
6306,
13,
198,
37811,
198,
8818,
923,
62,
23100,
3681,
7,
198,
220,
220,
220,
5456,
30642,
11,
6306,
30800,
7390,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
3419,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
32782,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
6800,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
360,
713,
90,
10100,
11,
7149,
92,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
16366,
30642,
1,
5218,
5456,
30642,
11,
366,
23100,
3681,
30800,
7390,
1,
5218,
6306,
30800,
7390,
198,
220,
220,
220,
220,
220,
220,
220,
5619,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
923,
62,
23100,
3681,
7,
198,
220,
220,
220,
5456,
30642,
11,
198,
220,
220,
220,
6306,
30800,
7390,
11,
198,
220,
220,
220,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
198,
220,
220,
220,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
22784,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
32782,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
6800,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
360,
713,
90,
10100,
11,
7149,
92,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4017,
39909,
342,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4808,
647,
469,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
360,
713,
90,
10100,
11,
7149,
92,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
16366,
30642,
1,
5218,
5456,
30642,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
23100,
3681,
30800,
7390,
1,
5218,
6306,
30800,
7390,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
10612,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
42287,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
10612,
198,
220,
220,
220,
220,
220,
220,
220,
5619,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
2245,
62,
23100,
3681,
7,
312,
8,
198,
220,
220,
220,
2245,
62,
23100,
3681,
7,
312,
11,
42287,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
1273,
2840,
262,
7368,
6306,
13,
198,
198,
2,
20559,
2886,
198,
12,
4600,
312,
63,
25,
383,
4522,
286,
262,
6306,
13,
198,
198,
37811,
198,
8818,
2245,
62,
23100,
3681,
7,
312,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
28955,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
7206,
2538,
9328,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
6800,
32624,
7,
312,
8,
8172,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
2245,
62,
23100,
3681,
7,
198,
220,
220,
220,
4686,
11,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
3419,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
7206,
2538,
9328,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
6800,
32624,
7,
312,
42501,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
26,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
7621,
62,
31092,
7,
31092,
62,
1501,
11,
15940,
8,
198,
220,
220,
220,
7621,
62,
31092,
7,
31092,
62,
1501,
11,
15940,
11,
42287,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
4677,
13508,
262,
7368,
15940,
284,
262,
7368,
8271,
13,
198,
198,
2,
20559,
2886,
198,
12,
4600,
31092,
62,
1501,
63,
25,
383,
6186,
20857,
6530,
357,
1503,
45,
8,
286,
262,
8271,
13,
198,
12,
4600,
31499,
63,
25,
383,
15940,
329,
262,
8271,
13,
198,
198,
37811,
198,
8818,
7621,
62,
31092,
7,
31092,
3163,
77,
11,
15940,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
28955,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
32782,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
31499,
32624,
7,
31092,
3163,
77,
42501,
198,
220,
220,
220,
220,
220,
220,
220,
360,
713,
90,
10100,
11,
7149,
92,
7203,
31499,
1,
5218,
15940,
1776,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
7621,
62,
31092,
7,
198,
220,
220,
220,
8271,
3163,
77,
11,
198,
220,
220,
220,
15940,
11,
198,
220,
220,
220,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
198,
220,
220,
220,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
22784,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
32782,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
31499,
32624,
7,
31092,
3163,
77,
42501,
198,
220,
220,
220,
220,
220,
220,
220,
360,
713,
90,
10100,
11,
7149,
92,
7,
647,
39909,
342,
28264,
647,
469,
11,
360,
713,
90,
10100,
11,
7149,
92,
7203,
31499,
1,
5218,
15940,
828,
42287,
18125,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
1418,
363,
62,
31092,
7,
31092,
62,
1501,
8,
198,
220,
220,
220,
1418,
363,
62,
31092,
7,
31092,
62,
1501,
11,
42287,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
8413,
5241,
262,
7368,
15940,
422,
262,
7368,
8271,
13,
198,
198,
2,
20559,
2886,
198,
12,
4600,
31092,
62,
1501,
63,
25,
383,
6186,
20857,
6530,
357,
1503,
45,
8,
286,
262,
8271,
13,
198,
198,
2,
32233,
40117,
198,
30719,
10007,
460,
307,
3804,
355,
257,
4600,
37266,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
92,
44646,
48951,
8251,
389,
25,
198,
12,
4600,
1,
12985,
40729,
1,
63,
25,
383,
7621,
8251,
284,
4781,
13,
198,
37811,
198,
8818,
1418,
363,
62,
31092,
7,
31092,
3163,
77,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
28955,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
7206,
2538,
9328,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
31499,
32624,
7,
31092,
3163,
77,
8,
8172,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
1418,
363,
62,
31092,
7,
198,
220,
220,
220,
8271,
3163,
77,
11,
198,
220,
220,
220,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
198,
220,
220,
220,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
22784,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
7206,
2538,
9328,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
31499,
32624,
7,
31092,
3163,
77,
42501,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
26,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
4296,
62,
23100,
3681,
62,
28243,
7,
312,
8,
198,
220,
220,
220,
4296,
62,
23100,
3681,
62,
28243,
7,
312,
11,
42287,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
30072,
198,
198,
4933,
19581,
262,
7368,
6306,
11055,
13,
198,
198,
2,
20559,
2886,
198,
12,
4600,
312,
63,
25,
383,
4522,
286,
262,
6306,
11055,
13,
198,
198,
2,
32233,
40117,
198,
30719,
10007,
460,
307,
3804,
355,
257,
4600,
37266,
3712,
35,
713,
90,
10100,
11,
27,
25,
7149,
92,
44646,
48951,
8251,
389,
25,
198,
12,
4600,
1,
4658,
1,
63,
25,
383,
4028,
329,
262,
6306,
13,
198,
12,
4600,
1,
11213,
1,
63,
25,
317,
6764,
329,
262,
11055,
13,
198,
12,
4600,
1,
18090,
3163,
77,
1,
63,
25,
383,
6186,
20857,
6530,
357,
1503,
45,
8,
286,
281,
314,
2390,
2597,
326,
11455,
262,
30865,
376,
1797,
198,
220,
2139,
7170,
284,
1620,
2139,
4028,
319,
534,
8378,
13,
198,
12,
4600,
1,
11338,
25559,
1756,
1,
63,
25,
383,
2245,
3403,
329,
262,
6306,
13,
198,
12,
4600,
1,
83,
853,
1039,
1,
63,
25,
383,
6670,
329,
262,
6306,
13,
198,
37811,
198,
8818,
4296,
62,
23100,
3681,
62,
28243,
7,
312,
26,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
28955,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
47,
11417,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
3681,
12966,
17041,
32624,
7,
312,
8,
8172,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198,
8818,
4296,
62,
23100,
3681,
62,
28243,
7,
198,
220,
220,
220,
4686,
11,
42287,
3712,
23839,
35,
713,
90,
10100,
19629,
3253,
82,
62,
11250,
3712,
23839,
12298,
50,
16934,
28,
20541,
62,
8356,
62,
11250,
3419,
198,
8,
198,
220,
220,
220,
1441,
277,
271,
7,
198,
220,
220,
220,
220,
220,
220,
220,
366,
47,
11417,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
12813,
23100,
3681,
12966,
17041,
32624,
7,
312,
42501,
198,
220,
220,
220,
220,
220,
220,
220,
42287,
26,
198,
220,
220,
220,
220,
220,
220,
220,
3253,
82,
62,
11250,
28,
8356,
62,
11250,
11,
198,
220,
220,
220,
220,
220,
220,
220,
3895,
62,
2617,
28,
35009,
27389,
62,
15112,
40086,
62,
28480,
11,
198,
220,
220,
220,
1267,
198,
437,
198
] | 2.525315 | 5,787 |
export fill_pbo!
# https://www.unavco.org/data/strain-seismic/bsm-data/lib/docs/bottle_format.pdf
bottle_chans = Dict{String, Tuple{String,String}}(
"BatteryVolts" => ("ABV", "V"),
"CalOffsetCH0G3" => ("AO0", "{unknown}"),
"CalOffsetCH1G3" => ("AO1", "{unknown}"),
"CalOffsetCH2G3" => ("AO2", "{unknown}"),
"CalOffsetCH3G3" => ("AO3", "{unknown}"),
"CalStepCH0G2" => ("A02", "{unknown}"),
"CalStepCH0G3" => ("A03", "{unknown}"),
"CalStepCH1G2" => ("A12", "{unknown}"),
"CalStepCH1G3" => ("A13", "{unknown}"),
"CalStepCH2G2" => ("A22", "{unknown}"),
"CalStepCH2G3" => ("A23", "{unknown}"),
"CalStepCH3G2" => ("A32", "{unknown}"),
"CalStepCH3G3" => ("A33", "{unknown}"),
"DownholeDegC" => ("KD", "Cel"),
"LoggerDegC" => ("K1", "Cel"),
"PowerBoxDegC" => ("K2", "Cel"),
"PressureKPa" => ("DI", "kPa"),
"Rainfallmm" => ("R0", "mm"),
"RTSettingCH0" => ("AR0", "{unknown}"),
"RTSettingCH1" => ("AR1", "{unknown}"),
"RTSettingCH2" => ("AR2", "{unknown}"),
"RTSettingCH3" => ("AR3", "{unknown}"),
"SolarAmps" => ("ASO", "A"),
"SystemAmps" => ("ASY", "A")
)
bottle_nets = Dict{String, String}(
"AIRS" => "MC",
"TEPE" => "GF",
"BUY1" => "GF",
"ESN1" => "GF",
"TRNT" => "MC",
"HALK" => "GF",
"B948" => "ARRA",
"OLV1" => "MC",
"OLV2" => "MC",
"GERD" => "MC",
"SIV1" => "GF",
"BOZ1" => "GF",
"B947" => "ARRA"
)
function check_bads!(x::AbstractArray, nv::T) where T
# Check for bad samples
@inbounds for i in x
if i == nv
return true
end
end
return false
end
function channel_guess(str::AbstractString, fs::Float64)
si = fs >= 1.0 ? 14 : 10
ei = length(str) - (endswith(str, "_20") ? 3 : 0)
# name, id, units
str = str[si:ei]
if length(str) == 3
units = "m/m"
else
(str, units) = get(bottle_chans, str, ("YY", "{unknown}"))
end
# form channel string
if length(str) == 2
str = (fs > 1.0 ? "B" : fs > 0.1 ? "L" : "R")*str
end
return (str, units)
end
function read_bottle!(S::GphysData, fstr::String, nx_new::Int64, nx_add::Int64, memmap::Bool, strict::Bool, v::Integer)
buf = BUF.buf
files = ls(fstr)
for file in files
io = memmap ? IOBuffer(Mmap.mmap(file)) : open(file, "r")
# Read header ============================================================
fastskip(io, 8)
t0 = round(Int64, fastread(io, Float64)*sμ)
dt = fastread(io, Float32)
nx = fastread(io, Int32)
ty = fastread(io, Int32)
nv = fastread(io, Int32)
fastskip(io, 8)
fs = 1.0/dt
v > 2 && println("t0 = ", t0, ", fs = ", fs, ", nx = ", nx, ", ty = ", ty, ", nv = ", nv)
# Read data ==============================================================
T = ty == 0 ? Int16 : ty == 1 ? Int32 : Float32
nb = nx*sizeof(T)
checkbuf_8!(buf, nb)
fast_readbytes!(io, buf, nb)
close(io)
# Try to create an ID from the file name =================================
# Assumes fname SSSSyyJJJ... (SSSS = station, yy = year, JJJ = Julian day)
fname = splitdir(file)[2]
sta = fname[1:4]
(cha, units) = channel_guess(fname, fs)
# find relevant entry in station data
net = get(bottle_nets, sta, "PB")
id = net * "." * sta * ".." * cha
# Load into S ============================================================
i = findid(id, S.id)
if strict
i = channel_match(S, i, fs)
end
if i == 0
# Create C.x
x = Array{Float64,1}(undef, max(nx_new, nx))
os = 1
C = SeisChannel()
setfield!(C, :id, id)
setfield!(C, :fs, fs)
setfield!(C, :units, units)
mk_t!(C, nx, t0)
setfield!(C, :x, x)
push!(S, C)
else
xi = S.t[i][end,1]
x = getindex(getfield(S, :x), i)
check_for_gap!(S, i, t0, nx, v)
Lx = length(x)
if xi + nx > Lx
resize!(x, Lx + max(nx_add, nx))
end
os = xi + 1
end
# Check for null values
nv = T(nv)
y = reinterpret(T, buf)
b = T == Int16 ? false : check_bads!(y, nv)
if b
j = os
@inbounds for i = 1:nx
if y[i] == nv
x[j] = NaN
else
x[j] = y[i]
end
j += 1
end
else
copyto!(x, os, y, 1, nx)
end
end
trunc_x!(S)
resize!(buf, 65535)
return nothing
end
function read_bottle(fstr::String, nx_new::Int64, nx_add::Int64, memmap::Bool, strict::Bool, v::Integer)
S = SeisData()
read_bottle!(S, fstr, nx_new, nx_add, memmap, strict, v)
return S
end
"""
fill_pbo!(S)
Attempt to fill `:name` and `:loc` fields of S using station names (second field of S.id) cross-referenced against a PBO station info file.
"""
function fill_pbo!(S::GphysData)
sta_data = readdlm(path * "/Formats/PBO_bsm_coords.txt", ',', comments=false)
sta_data[:,2] .= strip.(sta_data[:,2])
sta_data[:,6] .= strip.(sta_data[:,6])
n_sta = size(sta_data, 1)
for i = 1:S.n
sta = split(S.id[i], '.')[2]
for j = 1:n_sta
if sta_data[j, 1] == sta
S.name[i] = String(sta_data[j,2])
lat = sta_data[j,3]
lon = sta_data[j,4]
el = sta_data[j,5]
S.loc[i] = GeoLoc(lat = lat, lon = lon, el = el)
break
end
end
end
return nothing
end
| [
39344,
6070,
62,
79,
2127,
0,
198,
198,
2,
3740,
1378,
2503,
13,
403,
615,
1073,
13,
2398,
14,
7890,
14,
2536,
391,
12,
325,
1042,
291,
14,
1443,
76,
12,
7890,
14,
8019,
14,
31628,
14,
10985,
293,
62,
18982,
13,
12315,
198,
198,
10985,
293,
62,
354,
504,
796,
360,
713,
90,
10100,
11,
309,
29291,
90,
10100,
11,
10100,
11709,
7,
198,
220,
366,
47006,
16598,
912,
1,
5218,
5855,
6242,
53,
1600,
366,
53,
12340,
198,
220,
366,
9771,
34519,
3398,
15,
38,
18,
1,
5218,
5855,
32,
46,
15,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
9771,
34519,
3398,
16,
38,
18,
1,
5218,
5855,
32,
46,
16,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
9771,
34519,
3398,
17,
38,
18,
1,
5218,
5855,
32,
46,
17,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
9771,
34519,
3398,
18,
38,
18,
1,
5218,
5855,
32,
46,
18,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
9771,
8600,
3398,
15,
38,
17,
1,
5218,
5855,
32,
2999,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
9771,
8600,
3398,
15,
38,
18,
1,
5218,
5855,
32,
3070,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
9771,
8600,
3398,
16,
38,
17,
1,
5218,
5855,
32,
1065,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
9771,
8600,
3398,
16,
38,
18,
1,
5218,
5855,
32,
1485,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
9771,
8600,
3398,
17,
38,
17,
1,
5218,
5855,
32,
1828,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
9771,
8600,
3398,
17,
38,
18,
1,
5218,
5855,
32,
1954,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
9771,
8600,
3398,
18,
38,
17,
1,
5218,
5855,
32,
2624,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
9771,
8600,
3398,
18,
38,
18,
1,
5218,
5855,
32,
2091,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
8048,
13207,
35,
1533,
34,
1,
5218,
5855,
42,
35,
1600,
366,
34,
417,
12340,
198,
220,
366,
11187,
1362,
35,
1533,
34,
1,
5218,
5855,
42,
16,
1600,
366,
34,
417,
12340,
198,
220,
366,
13434,
14253,
35,
1533,
34,
1,
5218,
5855,
42,
17,
1600,
366,
34,
417,
12340,
198,
220,
366,
13800,
495,
42,
28875,
1,
5218,
5855,
17931,
1600,
366,
74,
28875,
12340,
198,
220,
366,
31443,
7207,
3020,
1,
5218,
5855,
49,
15,
1600,
366,
3020,
12340,
198,
220,
366,
49,
4694,
35463,
3398,
15,
1,
5218,
5855,
1503,
15,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
49,
4694,
35463,
3398,
16,
1,
5218,
5855,
1503,
16,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
49,
4694,
35463,
3398,
17,
1,
5218,
5855,
1503,
17,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
49,
4694,
35463,
3398,
18,
1,
5218,
5855,
1503,
18,
1600,
45144,
34680,
92,
12340,
198,
220,
366,
38825,
5840,
862,
1,
5218,
5855,
1921,
46,
1600,
366,
32,
12340,
198,
220,
366,
11964,
5840,
862,
1,
5218,
5855,
26483,
1600,
366,
32,
4943,
198,
8,
198,
198,
10985,
293,
62,
45938,
796,
360,
713,
90,
10100,
11,
10903,
92,
7,
198,
220,
366,
42149,
50,
1,
5218,
366,
9655,
1600,
198,
220,
366,
51,
8905,
36,
1,
5218,
366,
21713,
1600,
198,
220,
366,
19499,
56,
16,
1,
5218,
366,
21713,
1600,
198,
220,
366,
1546,
45,
16,
1,
5218,
366,
21713,
1600,
198,
220,
366,
5446,
11251,
1,
5218,
366,
9655,
1600,
198,
220,
366,
39,
28082,
1,
5218,
366,
21713,
1600,
198,
220,
366,
33,
24,
2780,
1,
5218,
366,
1503,
3861,
1600,
198,
220,
366,
3535,
53,
16,
1,
5218,
366,
9655,
1600,
198,
220,
366,
3535,
53,
17,
1,
5218,
366,
9655,
1600,
198,
220,
366,
30373,
35,
1,
5218,
366,
9655,
1600,
198,
220,
366,
50,
3824,
16,
1,
5218,
366,
21713,
1600,
198,
220,
366,
8202,
57,
16,
1,
5218,
366,
21713,
1600,
198,
220,
366,
33,
24,
2857,
1,
5218,
366,
1503,
3861,
1,
198,
220,
1267,
198,
198,
8818,
2198,
62,
65,
5643,
0,
7,
87,
3712,
23839,
19182,
11,
299,
85,
3712,
51,
8,
810,
309,
198,
220,
1303,
6822,
329,
2089,
8405,
198,
220,
2488,
259,
65,
3733,
329,
1312,
287,
2124,
198,
220,
220,
220,
611,
1312,
6624,
299,
85,
198,
220,
220,
220,
220,
220,
1441,
2081,
198,
220,
220,
220,
886,
198,
220,
886,
198,
220,
1441,
3991,
198,
437,
198,
198,
8818,
6518,
62,
5162,
408,
7,
2536,
3712,
23839,
10100,
11,
43458,
3712,
43879,
2414,
8,
198,
220,
33721,
796,
43458,
18189,
352,
13,
15,
5633,
1478,
1058,
838,
198,
220,
304,
72,
796,
4129,
7,
2536,
8,
532,
357,
437,
2032,
342,
7,
2536,
11,
45434,
1238,
4943,
5633,
513,
1058,
657,
8,
628,
220,
1303,
1438,
11,
4686,
11,
4991,
198,
220,
965,
796,
965,
58,
13396,
25,
20295,
60,
198,
220,
611,
4129,
7,
2536,
8,
6624,
513,
198,
220,
220,
220,
4991,
796,
366,
76,
14,
76,
1,
198,
220,
2073,
198,
220,
220,
220,
357,
2536,
11,
4991,
8,
796,
651,
7,
10985,
293,
62,
354,
504,
11,
965,
11,
5855,
26314,
1600,
45144,
34680,
36786,
4008,
198,
220,
886,
628,
220,
1303,
1296,
6518,
4731,
198,
220,
611,
4129,
7,
2536,
8,
6624,
362,
198,
220,
220,
220,
965,
796,
357,
9501,
1875,
352,
13,
15,
5633,
366,
33,
1,
1058,
43458,
1875,
657,
13,
16,
5633,
366,
43,
1,
1058,
366,
49,
4943,
9,
2536,
198,
220,
886,
628,
220,
1441,
357,
2536,
11,
4991,
8,
198,
437,
198,
198,
8818,
1100,
62,
10985,
293,
0,
7,
50,
3712,
38,
34411,
6601,
11,
277,
2536,
3712,
10100,
11,
299,
87,
62,
3605,
3712,
5317,
2414,
11,
299,
87,
62,
2860,
3712,
5317,
2414,
11,
1066,
8899,
3712,
33,
970,
11,
7646,
3712,
33,
970,
11,
410,
3712,
46541,
8,
198,
220,
42684,
796,
20571,
37,
13,
29325,
198,
220,
3696,
796,
43979,
7,
69,
2536,
8,
628,
220,
329,
2393,
287,
3696,
198,
220,
220,
220,
33245,
796,
1066,
8899,
5633,
314,
9864,
13712,
7,
44,
8899,
13,
3020,
499,
7,
7753,
4008,
1058,
1280,
7,
7753,
11,
366,
81,
4943,
628,
220,
220,
220,
1303,
4149,
13639,
46111,
4770,
2559,
18604,
198,
220,
220,
220,
3049,
48267,
7,
952,
11,
807,
8,
198,
220,
220,
220,
256,
15,
796,
2835,
7,
5317,
2414,
11,
3049,
961,
7,
952,
11,
48436,
2414,
27493,
82,
34703,
8,
198,
220,
220,
220,
288,
83,
796,
3049,
961,
7,
952,
11,
48436,
2624,
8,
198,
220,
220,
220,
299,
87,
796,
3049,
961,
7,
952,
11,
2558,
2624,
8,
198,
220,
220,
220,
1259,
796,
3049,
961,
7,
952,
11,
2558,
2624,
8,
198,
220,
220,
220,
299,
85,
796,
3049,
961,
7,
952,
11,
2558,
2624,
8,
198,
220,
220,
220,
3049,
48267,
7,
952,
11,
807,
8,
198,
220,
220,
220,
43458,
796,
352,
13,
15,
14,
28664,
198,
220,
220,
220,
410,
1875,
362,
11405,
44872,
7203,
83,
15,
796,
33172,
256,
15,
11,
33172,
43458,
796,
33172,
43458,
11,
33172,
299,
87,
796,
33172,
299,
87,
11,
33172,
1259,
796,
33172,
1259,
11,
33172,
299,
85,
796,
33172,
299,
85,
8,
628,
220,
220,
220,
1303,
4149,
1366,
46111,
4770,
25609,
28,
198,
220,
220,
220,
309,
796,
1259,
6624,
657,
5633,
2558,
1433,
1058,
1259,
6624,
352,
5633,
2558,
2624,
1058,
48436,
2624,
198,
220,
220,
220,
299,
65,
796,
299,
87,
9,
7857,
1659,
7,
51,
8,
198,
220,
220,
220,
2198,
29325,
62,
23,
0,
7,
29325,
11,
299,
65,
8,
198,
220,
220,
220,
3049,
62,
961,
33661,
0,
7,
952,
11,
42684,
11,
299,
65,
8,
198,
220,
220,
220,
1969,
7,
952,
8,
628,
220,
220,
220,
1303,
9993,
284,
2251,
281,
4522,
422,
262,
2393,
1438,
46111,
198,
220,
220,
220,
1303,
2195,
8139,
277,
3672,
311,
5432,
13940,
88,
32178,
41,
986,
357,
5432,
5432,
796,
4429,
11,
331,
88,
796,
614,
11,
449,
32178,
796,
18322,
1110,
8,
198,
220,
220,
220,
277,
3672,
796,
6626,
15908,
7,
7753,
38381,
17,
60,
198,
220,
220,
220,
336,
64,
796,
277,
3672,
58,
16,
25,
19,
60,
198,
220,
220,
220,
357,
11693,
11,
4991,
8,
796,
6518,
62,
5162,
408,
7,
69,
3672,
11,
43458,
8,
628,
220,
220,
220,
1303,
1064,
5981,
5726,
287,
4429,
1366,
198,
220,
220,
220,
2010,
796,
651,
7,
10985,
293,
62,
45938,
11,
336,
64,
11,
366,
49079,
4943,
198,
220,
220,
220,
4686,
796,
2010,
1635,
366,
526,
1635,
336,
64,
1635,
366,
492,
1,
1635,
17792,
628,
220,
220,
220,
1303,
8778,
656,
311,
46111,
4770,
2559,
18604,
198,
220,
220,
220,
1312,
796,
1064,
312,
7,
312,
11,
311,
13,
312,
8,
198,
220,
220,
220,
611,
7646,
198,
220,
220,
220,
220,
220,
1312,
796,
6518,
62,
15699,
7,
50,
11,
1312,
11,
43458,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
611,
1312,
6624,
657,
628,
220,
220,
220,
220,
220,
1303,
13610,
327,
13,
87,
198,
220,
220,
220,
220,
220,
2124,
796,
15690,
90,
43879,
2414,
11,
16,
92,
7,
917,
891,
11,
3509,
7,
77,
87,
62,
3605,
11,
299,
87,
4008,
198,
220,
220,
220,
220,
220,
28686,
796,
352,
628,
220,
220,
220,
220,
220,
327,
796,
1001,
271,
29239,
3419,
198,
220,
220,
220,
220,
220,
900,
3245,
0,
7,
34,
11,
1058,
312,
11,
4686,
8,
198,
220,
220,
220,
220,
220,
900,
3245,
0,
7,
34,
11,
1058,
9501,
11,
43458,
8,
198,
220,
220,
220,
220,
220,
900,
3245,
0,
7,
34,
11,
1058,
41667,
11,
4991,
8,
198,
220,
220,
220,
220,
220,
33480,
62,
83,
0,
7,
34,
11,
299,
87,
11,
256,
15,
8,
198,
220,
220,
220,
220,
220,
900,
3245,
0,
7,
34,
11,
1058,
87,
11,
2124,
8,
198,
220,
220,
220,
220,
220,
4574,
0,
7,
50,
11,
327,
8,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
2124,
72,
796,
311,
13,
83,
58,
72,
7131,
437,
11,
16,
60,
198,
220,
220,
220,
220,
220,
2124,
796,
651,
9630,
7,
1136,
3245,
7,
50,
11,
1058,
87,
828,
1312,
8,
198,
220,
220,
220,
220,
220,
2198,
62,
1640,
62,
43554,
0,
7,
50,
11,
1312,
11,
256,
15,
11,
299,
87,
11,
410,
8,
198,
220,
220,
220,
220,
220,
406,
87,
796,
4129,
7,
87,
8,
198,
220,
220,
220,
220,
220,
611,
2124,
72,
1343,
299,
87,
1875,
406,
87,
198,
220,
220,
220,
220,
220,
220,
220,
47558,
0,
7,
87,
11,
406,
87,
1343,
3509,
7,
77,
87,
62,
2860,
11,
299,
87,
4008,
198,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
28686,
796,
2124,
72,
1343,
352,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
6822,
329,
9242,
3815,
198,
220,
220,
220,
299,
85,
796,
309,
7,
48005,
8,
198,
220,
220,
220,
331,
796,
302,
27381,
7,
51,
11,
42684,
8,
198,
220,
220,
220,
275,
796,
309,
6624,
2558,
1433,
5633,
3991,
1058,
2198,
62,
65,
5643,
0,
7,
88,
11,
299,
85,
8,
198,
220,
220,
220,
611,
275,
198,
220,
220,
220,
220,
220,
474,
796,
28686,
198,
220,
220,
220,
220,
220,
2488,
259,
65,
3733,
329,
1312,
796,
352,
25,
77,
87,
198,
220,
220,
220,
220,
220,
220,
220,
611,
331,
58,
72,
60,
6624,
299,
85,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
58,
73,
60,
796,
11013,
45,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
58,
73,
60,
796,
331,
58,
72,
60,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
474,
15853,
352,
198,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
4866,
1462,
0,
7,
87,
11,
28686,
11,
331,
11,
352,
11,
299,
87,
8,
198,
220,
220,
220,
886,
198,
220,
886,
198,
220,
40122,
62,
87,
0,
7,
50,
8,
198,
220,
47558,
0,
7,
29325,
11,
45021,
2327,
8,
628,
220,
1441,
2147,
198,
437,
198,
198,
8818,
1100,
62,
10985,
293,
7,
69,
2536,
3712,
10100,
11,
299,
87,
62,
3605,
3712,
5317,
2414,
11,
299,
87,
62,
2860,
3712,
5317,
2414,
11,
1066,
8899,
3712,
33,
970,
11,
7646,
3712,
33,
970,
11,
410,
3712,
46541,
8,
628,
220,
311,
796,
1001,
271,
6601,
3419,
198,
220,
1100,
62,
10985,
293,
0,
7,
50,
11,
277,
2536,
11,
299,
87,
62,
3605,
11,
299,
87,
62,
2860,
11,
1066,
8899,
11,
7646,
11,
410,
8,
198,
220,
1441,
311,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
6070,
62,
79,
2127,
0,
7,
50,
8,
198,
198,
37177,
284,
6070,
4600,
25,
3672,
63,
290,
4600,
25,
17946,
63,
7032,
286,
311,
1262,
4429,
3891,
357,
12227,
2214,
286,
311,
13,
312,
8,
3272,
12,
5420,
14226,
771,
1028,
257,
350,
8202,
4429,
7508,
2393,
13,
198,
37811,
198,
8818,
6070,
62,
79,
2127,
0,
7,
50,
3712,
38,
34411,
6601,
8,
198,
220,
336,
64,
62,
7890,
796,
1100,
25404,
76,
7,
6978,
1635,
12813,
8479,
1381,
14,
47,
8202,
62,
1443,
76,
62,
1073,
3669,
13,
14116,
1600,
46083,
3256,
3651,
28,
9562,
8,
198,
220,
336,
64,
62,
7890,
58,
45299,
17,
60,
764,
28,
10283,
12195,
38031,
62,
7890,
58,
45299,
17,
12962,
198,
220,
336,
64,
62,
7890,
58,
45299,
21,
60,
764,
28,
10283,
12195,
38031,
62,
7890,
58,
45299,
21,
12962,
198,
220,
299,
62,
38031,
796,
2546,
7,
38031,
62,
7890,
11,
352,
8,
198,
220,
329,
1312,
796,
352,
25,
50,
13,
77,
198,
220,
220,
220,
336,
64,
796,
6626,
7,
50,
13,
312,
58,
72,
4357,
705,
2637,
38381,
17,
60,
198,
220,
220,
220,
329,
474,
796,
352,
25,
77,
62,
38031,
198,
220,
220,
220,
220,
220,
611,
336,
64,
62,
7890,
58,
73,
11,
352,
60,
6624,
336,
64,
198,
220,
220,
220,
220,
220,
220,
220,
311,
13,
3672,
58,
72,
60,
796,
10903,
7,
38031,
62,
7890,
58,
73,
11,
17,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
3042,
796,
336,
64,
62,
7890,
58,
73,
11,
18,
60,
198,
220,
220,
220,
220,
220,
220,
220,
300,
261,
796,
336,
64,
62,
7890,
58,
73,
11,
19,
60,
198,
220,
220,
220,
220,
220,
220,
220,
1288,
796,
336,
64,
62,
7890,
58,
73,
11,
20,
60,
198,
220,
220,
220,
220,
220,
220,
220,
311,
13,
17946,
58,
72,
60,
796,
32960,
33711,
7,
15460,
796,
3042,
11,
300,
261,
796,
300,
261,
11,
1288,
796,
1288,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2270,
198,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
886,
198,
220,
1441,
2147,
198,
437,
198
] | 2.093285 | 2,487 |
module FisherPlot
using LaTeXStrings
using CairoMakie
using Makie
using Base: @kwdef
function ellipseparameterization(a::Float64, b::Float64, θ::Float64)
t = LinRange(0,2π, 200)
x = Array(a .* sin.(θ) .* cos.(t) + b .* cos.(θ) .* sin.(t))
y = Array(a .* cos.(θ) .* cos.(t) - b .* sin.(θ) .* sin.(t))
return x, y
end
function gaussian(μ::Float64, σ::Float64, x)
return 1/(sqrt(2π*σ^2))*exp(-0.5*(x-μ)^2/σ^2)
end
function ellipseparameters(covmatrix::Matrix{Float64}, i::Int64, j::Int64)
σi = sqrt(covmatrix[i,i])
σj = sqrt(covmatrix[j,j])
σij = covmatrix[i,j]
θ = (atan(2σij,(σi^2-σj^2)))/2
a = sqrt((σi^2+σj^2)/2+sqrt(((σi^2-σj^2)^2)/4+σij^2))
if i == j
b = 0.
else
b = sqrt((σi^2+σj^2)/2-sqrt(((σi^2-σj^2)^2)/4+σij^2))
end
return σi, σj, a, b, θ
end
function preparecanvas(LaTeX_array, limits, ticks, probes, colors, PlotPars::Dict)
matrix_dim = length(LaTeX_array)
#TODO: add the textsize to PlotPars
figure = Makie.Figure(textsize = 40, font = PlotPars["font"])
for i in 1:matrix_dim
for j in 1:i
if i == j
ax = Axis(figure[i,i],
width = PlotPars["sidesquare"], height = PlotPars["sidesquare"],
xticklabelsize = PlotPars["dimticklabel"],
yticklabelsize = PlotPars["dimticklabel"], yaxisposition = (:right),
xlabel = L"%$((LaTeX_array)[i])", xlabelsize = PlotPars["parslabelsize"],
ylabel = L"P/P_\mathrm{max}",ylabelsize = PlotPars["PPmaxlabelsize"], yticks = [0,1],
xticklabelrotation = PlotPars["xticklabelrotation"],
xticks = ([ticks[i,1], 0.5*(ticks[i,1]+ticks[i,2]), ticks[i,2]],
[string(myi) for myi in round.([ticks[i,1], 0.5*(ticks[i,1]+ticks[i,2]), ticks[i,2]], sigdigits = 3)]))
Makie.ylims!(ax, (-0.0,1.05))
Makie.xlims!(ax, (limits[i,1],limits[i,2]))
Makie.hideydecorations!(ax, ticks = false, ticklabels = false, label = false)
if i != matrix_dim
ax.alignmode = Mixed(right = MakieLayout.Protrusion(0), bottom = MakieLayout.Protrusion(0), top= MakieLayout.Protrusion(0))
hidexdecorations!(ax, ticks = true, ticklabels = true, label = true)
else
hidexdecorations!(ax, ticks = false, ticklabels = false, label = false)
end
else
ax = Axis(figure[i,j], width = PlotPars["sidesquare"], height = PlotPars["sidesquare"],
xticklabelsize = PlotPars["dimticklabel"], yticklabelsize = PlotPars["dimticklabel"],
ylabel = L"%$(LaTeX_array[i])", xlabel = L"%$((LaTeX_array)[j])",
ylabelsize = PlotPars["parslabelsize"], xlabelsize = PlotPars["parslabelsize"], xticklabelrotation = PlotPars["xticklabelrotation"],
yticks = ([ticks[i,1], 0.5*(ticks[i,1]+ticks[i,2]), ticks[i,2]],
[string(myi) for myi in round.([ticks[i,1], 0.5*(ticks[i,1]+ticks[i,2]), ticks[i,2]], sigdigits = 3)]),
xticks = ([ticks[j,1], 0.5*(ticks[j,1]+ticks[j,2]), ticks[j,2]],
[string(myi) for myi in round.([ticks[j,1], 0.5*(ticks[j,1]+ticks[j,2]), ticks[j,2]], sigdigits = 3)]),
yticklabelpad=8)
Makie.ylims!(ax, (limits[i,1],limits[i,2]))
Makie.xlims!(ax, (limits[j,1],limits[j,2]))
if i == matrix_dim
hidexdecorations!(ax, ticks = false, ticklabels = false, label = false)
else
hidexdecorations!(ax, ticks = true, ticklabels = true, label = true)
end
if j == 1
hideydecorations!(ax, ticks = false, ticklabels = false, label = false)
Legend(figure[1,matrix_dim],
[PolyElement(color = color, strokecolor = color, strokewidth = 1) for color in colors],
probes,
tellheight = false, tellwidth = false, rowgap = 10,
halign = :right, valign = :top, framecolor = :black, labelsize =55, patchsize = (70, 40), framevisible = true)
else
hideydecorations!(ax, ticks = true, ticklabels = true, label = true)
ax.alignmode = Mixed(right = MakieLayout.Protrusion(0), bottom = MakieLayout.Protrusion(0), top = MakieLayout.Protrusion(0))
end
end
end
end
Makie.resize!(figure.scene, figure.layout.layoutobservables.reportedsize[]...)
return figure
end
function drawgaussian!(canvas, σ, i, central, color)
ax = canvas[i,i]
x = Array(LinRange(-4σ+central,4σ+central, 200))
Makie.lines!(ax, x, gaussian.(central, σ, x)./gaussian.(central, σ, central), color = color, linewidth = 4)
Makie.band!(ax, x, 0, gaussian.(central, σ, x)./gaussian.(central, σ, central) , color=(color, 0.2))
x = Array(LinRange(-σ+central,σ+central, 200))
Makie.band!(ax, x, 0, gaussian.(central, σ, x)./gaussian.(central, σ, central) , color=(color, 0.4))
end
function drawellipse!(canvas, i, j, x, y, central_values, color)
ax = canvas[i,j]
Makie.lines!(ax, x .+ central_values[j], y .+ central_values[i], color = color, linewidth = 4)
Makie.lines!(ax, 3x .+ central_values[j], 3y .+ central_values[i], color = color, linewidth = 4)
Makie.band!(ax, x .+ central_values[j], 0, y .+ central_values[i], color=(color, 0.4))
Makie.band!(ax, 3x .+ central_values[j], 0, 3y .+ central_values[i], color=(color, 0.2))
end
function paintcorrmatrix!(canvas, central_values, corr_matrix, color)
for i in 1:length(central_values)
for j in 1:i
if i == j
drawgaussian!(canvas, sqrt(corr_matrix[i,i]), i, central_values[i], color)
else
σi, σj, a, b, θ = ellipseparameters(corr_matrix, i,j)
x,y = ellipseparameterization(a, b, θ)
drawellipse!(canvas, i, j, x, y, central_values, color)
end
end
end
end
function save(filename, obj)
Makie.save(filename, obj)
end
end # module
| [
21412,
14388,
43328,
198,
198,
3500,
4689,
49568,
13290,
654,
198,
3500,
23732,
44,
461,
494,
198,
3500,
15841,
494,
198,
3500,
7308,
25,
2488,
46265,
4299,
198,
198,
8818,
30004,
541,
325,
17143,
2357,
1634,
7,
64,
3712,
43879,
2414,
11,
275,
3712,
43879,
2414,
11,
7377,
116,
3712,
43879,
2414,
8,
198,
220,
220,
220,
256,
796,
5164,
17257,
7,
15,
11,
17,
46582,
11,
939,
8,
198,
220,
220,
220,
2124,
796,
15690,
7,
64,
764,
9,
7813,
12195,
138,
116,
8,
764,
9,
8615,
12195,
83,
8,
1343,
275,
764,
9,
8615,
12195,
138,
116,
8,
764,
9,
7813,
12195,
83,
4008,
198,
220,
220,
220,
331,
796,
15690,
7,
64,
764,
9,
8615,
12195,
138,
116,
8,
764,
9,
8615,
12195,
83,
8,
532,
275,
764,
9,
7813,
12195,
138,
116,
8,
764,
9,
7813,
12195,
83,
4008,
198,
220,
220,
220,
1441,
2124,
11,
331,
198,
437,
198,
198,
8818,
31986,
31562,
7,
34703,
3712,
43879,
2414,
11,
18074,
225,
3712,
43879,
2414,
11,
2124,
8,
198,
220,
220,
220,
1441,
352,
29006,
31166,
17034,
7,
17,
46582,
9,
38392,
61,
17,
4008,
9,
11201,
32590,
15,
13,
20,
9,
7,
87,
12,
34703,
8,
61,
17,
14,
38392,
61,
17,
8,
198,
437,
198,
198,
8818,
30004,
541,
325,
17143,
7307,
7,
66,
709,
6759,
8609,
3712,
46912,
90,
43879,
2414,
5512,
1312,
3712,
5317,
2414,
11,
474,
3712,
5317,
2414,
8,
198,
220,
220,
220,
18074,
225,
72,
796,
19862,
17034,
7,
66,
709,
6759,
8609,
58,
72,
11,
72,
12962,
198,
220,
220,
220,
18074,
225,
73,
796,
19862,
17034,
7,
66,
709,
6759,
8609,
58,
73,
11,
73,
12962,
198,
220,
220,
220,
18074,
225,
2926,
796,
39849,
6759,
8609,
58,
72,
11,
73,
60,
198,
220,
220,
220,
7377,
116,
796,
357,
39036,
7,
17,
38392,
2926,
11,
7,
38392,
72,
61,
17,
12,
38392,
73,
61,
17,
4008,
20679,
17,
198,
220,
220,
220,
257,
796,
19862,
17034,
19510,
38392,
72,
61,
17,
10,
38392,
73,
61,
17,
20679,
17,
10,
31166,
17034,
19510,
7,
38392,
72,
61,
17,
12,
38392,
73,
61,
17,
8,
61,
17,
20679,
19,
10,
38392,
2926,
61,
17,
4008,
198,
220,
220,
220,
611,
1312,
6624,
474,
198,
220,
220,
220,
220,
220,
220,
220,
275,
796,
657,
13,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
275,
796,
19862,
17034,
19510,
38392,
72,
61,
17,
10,
38392,
73,
61,
17,
20679,
17,
12,
31166,
17034,
19510,
7,
38392,
72,
61,
17,
12,
38392,
73,
61,
17,
8,
61,
17,
20679,
19,
10,
38392,
2926,
61,
17,
4008,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
18074,
225,
72,
11,
18074,
225,
73,
11,
257,
11,
275,
11,
7377,
116,
198,
437,
198,
198,
8818,
8335,
5171,
11017,
7,
14772,
49568,
62,
18747,
11,
7095,
11,
36066,
11,
33124,
11,
7577,
11,
28114,
47,
945,
3712,
35,
713,
8,
198,
220,
220,
220,
17593,
62,
27740,
796,
4129,
7,
14772,
49568,
62,
18747,
8,
198,
220,
220,
220,
1303,
51,
3727,
46,
25,
751,
262,
2420,
7857,
284,
28114,
47,
945,
198,
220,
220,
220,
3785,
796,
15841,
494,
13,
11337,
7,
5239,
7857,
796,
2319,
11,
10369,
796,
28114,
47,
945,
14692,
10331,
8973,
8,
198,
220,
220,
220,
329,
1312,
287,
352,
25,
6759,
8609,
62,
27740,
198,
220,
220,
220,
220,
220,
220,
220,
329,
474,
287,
352,
25,
72,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
1312,
6624,
474,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7877,
796,
38349,
7,
26875,
58,
72,
11,
72,
4357,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
9647,
796,
28114,
47,
945,
14692,
82,
1460,
421,
533,
33116,
6001,
796,
28114,
47,
945,
14692,
82,
1460,
421,
533,
33116,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
742,
624,
23912,
1424,
1096,
796,
28114,
47,
945,
14692,
27740,
42298,
18242,
33116,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
331,
42298,
23912,
1424,
1096,
796,
28114,
47,
945,
14692,
27740,
42298,
18242,
33116,
331,
22704,
9150,
796,
357,
25,
3506,
828,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
18242,
796,
406,
1,
4,
3,
19510,
14772,
49568,
62,
18747,
38381,
72,
12962,
1600,
2124,
23912,
1424,
1096,
796,
28114,
47,
945,
14692,
79,
945,
23912,
1424,
1096,
33116,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
331,
18242,
796,
406,
1,
47,
14,
47,
62,
59,
11018,
26224,
90,
9806,
92,
1600,
2645,
397,
1424,
1096,
796,
28114,
47,
945,
14692,
10246,
9806,
23912,
1424,
1096,
33116,
331,
83,
3378,
796,
685,
15,
11,
16,
4357,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
742,
624,
18242,
10599,
341,
796,
28114,
47,
945,
14692,
742,
624,
18242,
10599,
341,
33116,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
742,
3378,
796,
29565,
83,
3378,
58,
72,
11,
16,
4357,
657,
13,
20,
9,
7,
83,
3378,
58,
72,
11,
16,
48688,
83,
3378,
58,
72,
11,
17,
46570,
36066,
58,
72,
11,
17,
60,
4357,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
685,
8841,
7,
1820,
72,
8,
329,
616,
72,
287,
2835,
12195,
58,
83,
3378,
58,
72,
11,
16,
4357,
657,
13,
20,
9,
7,
83,
3378,
58,
72,
11,
16,
48688,
83,
3378,
58,
72,
11,
17,
46570,
36066,
58,
72,
11,
17,
60,
4357,
43237,
12894,
896,
796,
513,
15437,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
15841,
494,
13,
88,
2475,
82,
0,
7,
897,
11,
13841,
15,
13,
15,
11,
16,
13,
2713,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
15841,
494,
13,
87,
2475,
82,
0,
7,
897,
11,
357,
49196,
58,
72,
11,
16,
4357,
49196,
58,
72,
11,
17,
60,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
15841,
494,
13,
24717,
5173,
721,
273,
602,
0,
7,
897,
11,
36066,
796,
3991,
11,
4378,
23912,
1424,
796,
3991,
11,
6167,
796,
3991,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
1312,
14512,
17593,
62,
27740,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7877,
13,
31494,
14171,
796,
35250,
7,
3506,
796,
15841,
494,
32517,
13,
2964,
2213,
4241,
7,
15,
828,
4220,
796,
15841,
494,
32517,
13,
2964,
2213,
4241,
7,
15,
828,
1353,
28,
15841,
494,
32517,
13,
2964,
2213,
4241,
7,
15,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7808,
87,
12501,
273,
602,
0,
7,
897,
11,
36066,
796,
2081,
11,
4378,
23912,
1424,
796,
2081,
11,
220,
6167,
796,
2081,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7808,
87,
12501,
273,
602,
0,
7,
897,
11,
36066,
796,
3991,
11,
4378,
23912,
1424,
796,
3991,
11,
220,
6167,
796,
3991,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7877,
796,
38349,
7,
26875,
58,
72,
11,
73,
4357,
9647,
796,
28114,
47,
945,
14692,
82,
1460,
421,
533,
33116,
6001,
796,
28114,
47,
945,
14692,
82,
1460,
421,
533,
33116,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
742,
624,
23912,
1424,
1096,
796,
28114,
47,
945,
14692,
27740,
42298,
18242,
33116,
331,
42298,
23912,
1424,
1096,
796,
28114,
47,
945,
14692,
27740,
42298,
18242,
33116,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
331,
18242,
796,
406,
1,
4,
3,
7,
14772,
49568,
62,
18747,
58,
72,
12962,
1600,
2124,
18242,
796,
406,
1,
4,
3,
19510,
14772,
49568,
62,
18747,
38381,
73,
12962,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
331,
23912,
1424,
1096,
796,
28114,
47,
945,
14692,
79,
945,
23912,
1424,
1096,
33116,
2124,
23912,
1424,
1096,
796,
28114,
47,
945,
14692,
79,
945,
23912,
1424,
1096,
33116,
220,
742,
624,
18242,
10599,
341,
796,
28114,
47,
945,
14692,
742,
624,
18242,
10599,
341,
33116,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
331,
83,
3378,
796,
29565,
83,
3378,
58,
72,
11,
16,
4357,
657,
13,
20,
9,
7,
83,
3378,
58,
72,
11,
16,
48688,
83,
3378,
58,
72,
11,
17,
46570,
36066,
58,
72,
11,
17,
60,
4357,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
685,
8841,
7,
1820,
72,
8,
329,
616,
72,
287,
2835,
12195,
58,
83,
3378,
58,
72,
11,
16,
4357,
657,
13,
20,
9,
7,
83,
3378,
58,
72,
11,
16,
48688,
83,
3378,
58,
72,
11,
17,
46570,
36066,
58,
72,
11,
17,
60,
4357,
43237,
12894,
896,
796,
513,
15437,
828,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
742,
3378,
796,
29565,
83,
3378,
58,
73,
11,
16,
4357,
657,
13,
20,
9,
7,
83,
3378,
58,
73,
11,
16,
48688,
83,
3378,
58,
73,
11,
17,
46570,
36066,
58,
73,
11,
17,
60,
4357,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
685,
8841,
7,
1820,
72,
8,
329,
616,
72,
287,
2835,
12195,
58,
83,
3378,
58,
73,
11,
16,
4357,
657,
13,
20,
9,
7,
83,
3378,
58,
73,
11,
16,
48688,
83,
3378,
58,
73,
11,
17,
46570,
36066,
58,
73,
11,
17,
60,
4357,
43237,
12894,
896,
796,
513,
15437,
828,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
331,
42298,
18242,
15636,
28,
23,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
15841,
494,
13,
88,
2475,
82,
0,
7,
897,
11,
357,
49196,
58,
72,
11,
16,
4357,
49196,
58,
72,
11,
17,
60,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
15841,
494,
13,
87,
2475,
82,
0,
7,
897,
11,
357,
49196,
58,
73,
11,
16,
4357,
49196,
58,
73,
11,
17,
60,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
1312,
6624,
17593,
62,
27740,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7808,
87,
12501,
273,
602,
0,
7,
897,
11,
36066,
796,
3991,
11,
4378,
23912,
1424,
796,
3991,
11,
220,
6167,
796,
3991,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7808,
87,
12501,
273,
602,
0,
7,
897,
11,
36066,
796,
2081,
11,
4378,
23912,
1424,
796,
2081,
11,
220,
6167,
796,
2081,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
474,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7808,
5173,
721,
273,
602,
0,
7,
897,
11,
36066,
796,
3991,
11,
4378,
23912,
1424,
796,
3991,
11,
220,
6167,
796,
3991,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
9883,
7,
26875,
58,
16,
11,
6759,
8609,
62,
27740,
4357,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
685,
34220,
20180,
7,
8043,
796,
3124,
11,
14000,
8043,
796,
3124,
11,
14000,
10394,
796,
352,
8,
329,
3124,
287,
7577,
4357,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
33124,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1560,
17015,
796,
3991,
11,
1560,
10394,
796,
3991,
11,
5752,
43554,
796,
838,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
10284,
570,
796,
1058,
3506,
11,
1188,
570,
796,
1058,
4852,
11,
5739,
8043,
796,
1058,
13424,
11,
14722,
1096,
796,
2816,
11,
8529,
7857,
796,
357,
2154,
11,
2319,
828,
5739,
23504,
796,
2081,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7808,
5173,
721,
273,
602,
0,
7,
897,
11,
36066,
796,
2081,
11,
4378,
23912,
1424,
796,
2081,
11,
220,
6167,
796,
2081,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7877,
13,
31494,
14171,
796,
35250,
7,
3506,
796,
15841,
494,
32517,
13,
2964,
2213,
4241,
7,
15,
828,
4220,
796,
15841,
494,
32517,
13,
2964,
2213,
4241,
7,
15,
828,
1353,
796,
15841,
494,
32517,
13,
2964,
2213,
4241,
7,
15,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
15841,
494,
13,
411,
1096,
0,
7,
26875,
13,
29734,
11,
3785,
13,
39786,
13,
39786,
672,
3168,
2977,
13,
13116,
5379,
1096,
21737,
23029,
198,
220,
220,
220,
1441,
3785,
198,
437,
198,
198,
8818,
3197,
4908,
31562,
0,
7,
5171,
11017,
11,
18074,
225,
11,
1312,
11,
4318,
11,
3124,
8,
198,
220,
220,
220,
7877,
796,
21978,
58,
72,
11,
72,
60,
198,
220,
220,
220,
2124,
796,
15690,
7,
14993,
17257,
32590,
19,
38392,
10,
31463,
11,
19,
38392,
10,
31463,
11,
939,
4008,
198,
220,
220,
220,
15841,
494,
13,
6615,
0,
7,
897,
11,
2124,
11,
31986,
31562,
12195,
31463,
11,
18074,
225,
11,
2124,
737,
14,
4908,
31562,
12195,
31463,
11,
18074,
225,
11,
4318,
828,
3124,
796,
3124,
11,
9493,
413,
5649,
796,
604,
8,
198,
220,
220,
220,
15841,
494,
13,
3903,
0,
7,
897,
11,
2124,
11,
657,
11,
31986,
31562,
12195,
31463,
11,
18074,
225,
11,
2124,
737,
14,
4908,
31562,
12195,
31463,
11,
18074,
225,
11,
4318,
8,
837,
3124,
16193,
8043,
11,
657,
13,
17,
4008,
198,
220,
220,
220,
2124,
796,
15690,
7,
14993,
17257,
32590,
38392,
10,
31463,
11,
38392,
10,
31463,
11,
939,
4008,
198,
220,
220,
220,
15841,
494,
13,
3903,
0,
7,
897,
11,
2124,
11,
657,
11,
31986,
31562,
12195,
31463,
11,
18074,
225,
11,
2124,
737,
14,
4908,
31562,
12195,
31463,
11,
18074,
225,
11,
4318,
8,
837,
3124,
16193,
8043,
11,
657,
13,
19,
4008,
198,
437,
198,
198,
8818,
3197,
695,
541,
325,
0,
7,
5171,
11017,
11,
1312,
11,
474,
11,
2124,
11,
331,
11,
4318,
62,
27160,
11,
3124,
8,
198,
220,
220,
220,
7877,
796,
21978,
58,
72,
11,
73,
60,
198,
220,
220,
220,
220,
198,
220,
220,
220,
15841,
494,
13,
6615,
0,
7,
897,
11,
2124,
764,
10,
4318,
62,
27160,
58,
73,
4357,
331,
764,
10,
4318,
62,
27160,
58,
72,
4357,
3124,
796,
3124,
11,
9493,
413,
5649,
796,
604,
8,
198,
220,
220,
220,
15841,
494,
13,
6615,
0,
7,
897,
11,
513,
87,
764,
10,
4318,
62,
27160,
58,
73,
4357,
513,
88,
764,
10,
4318,
62,
27160,
58,
72,
4357,
3124,
796,
3124,
11,
9493,
413,
5649,
796,
604,
8,
628,
220,
220,
220,
15841,
494,
13,
3903,
0,
7,
897,
11,
2124,
764,
10,
4318,
62,
27160,
58,
73,
4357,
657,
11,
331,
764,
10,
4318,
62,
27160,
58,
72,
4357,
3124,
16193,
8043,
11,
657,
13,
19,
4008,
198,
220,
220,
220,
15841,
494,
13,
3903,
0,
7,
897,
11,
513,
87,
764,
10,
4318,
62,
27160,
58,
73,
4357,
657,
11,
513,
88,
764,
10,
4318,
62,
27160,
58,
72,
4357,
3124,
16193,
8043,
11,
657,
13,
17,
4008,
198,
437,
198,
198,
8818,
7521,
10215,
81,
6759,
8609,
0,
7,
5171,
11017,
11,
4318,
62,
27160,
11,
1162,
81,
62,
6759,
8609,
11,
3124,
8,
198,
220,
220,
220,
329,
1312,
287,
352,
25,
13664,
7,
31463,
62,
27160,
8,
198,
220,
220,
220,
220,
220,
220,
220,
329,
474,
287,
352,
25,
72,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
1312,
6624,
474,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3197,
4908,
31562,
0,
7,
5171,
11017,
11,
19862,
17034,
7,
10215,
81,
62,
6759,
8609,
58,
72,
11,
72,
46570,
1312,
11,
4318,
62,
27160,
58,
72,
4357,
3124,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
18074,
225,
72,
11,
18074,
225,
73,
11,
257,
11,
275,
11,
7377,
116,
796,
30004,
541,
325,
17143,
7307,
7,
10215,
81,
62,
6759,
8609,
11,
1312,
11,
73,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
11,
88,
796,
30004,
541,
325,
17143,
2357,
1634,
7,
64,
11,
275,
11,
7377,
116,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3197,
695,
541,
325,
0,
7,
5171,
11017,
11,
1312,
11,
474,
11,
2124,
11,
331,
11,
4318,
62,
27160,
11,
3124,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
3613,
7,
34345,
11,
26181,
8,
198,
220,
220,
220,
15841,
494,
13,
21928,
7,
34345,
11,
26181,
8,
198,
437,
198,
198,
437,
1303,
8265,
198
] | 1.913136 | 3,281 |
struct BinaryRelation{O, S, T}
x::S
y::T
end
BinaryRelation{O}(x::X, y::Y) where {O,X,Y} = BinaryRelation{O,X,Y}(x, y)
x = DiscreteVariable(:x, 1:5)
y = DiscreteVariable(:y, 2:6)
# BinaryRelation(==, x, y)
# BinaryRelation(==, x, 1)
# Use NodeVariables to break up binary relations like x + y ~ 3 and ternary such as x + y <= z
struct NodeVariable <: Var
name
op
x
y
domain
booleans
varmap
end
Base.show(io::IO, var::NodeVariable) = print(io, "$(var.name) = $(var.op)($(var.x), $(var.y))")
find_domain(op, x, y) = sort(collect(Set([op(i, j) for i in domain(x) for j in domain(y)])))
domain(v::Var) = v.domain
booleans(v::Var) = v.booleans
domain(x::Real) = x:x
"All booleans inside an expression that must be added to the encoding"
recursive_booleans(v::NodeVariable) = v.booleans ∪ booleans(v.x) ∪ booleans(v.y)
function NodeVariable(op, x, y, name=gensym())
domain = find_domain(op, x, y)
booleans = [Variable(name, i) for i ∈ indices(domain)]
varmap = Dict(i => v for (i, v) in zip(domain, booleans))
NodeVariable(name, op, x, y, domain, booleans, varmap)
end
Base.:+(x::Var, y) = NodeVariable(+, x, y)
Base.:+(x, y::Var) = NodeVariable(+, x, y)
Base.:*(x::Var, y) = NodeVariable(*, x, y)
Base.:*(x, y::Var) = NodeVariable(*, x, y)
Base.:^(x::Var, y) = NodeVariable(^, x, y)
Base.:^(x, y::Var) = NodeVariable(^, x, y)
function clauses(var::NodeVariable)
op = var.op
x = var.x
y = var.y
clauses = exactly_one(var.booleans)
# deal with 2 + X
if x isa Real
for j in domain(var.y)
value = op(x, j)
# y == j => var == op(x, j)
push!(clauses, ¬(y.varmap[j]) ∨ var.varmap[value])
end
# deal with x + 2
elseif y isa Real
for i in domain(var.x)
value = op(i, y)
# x == i => var == op(i, y)
push!(clauses, ¬(x.varmap[i]) ∨ var.varmap[value])
end
else
# neither x nor y is real
for i in domain(var.x)
for j in domain(var.y)
value = op(i, j)
# x == i && y == j => var == op(i, j)
push!(clauses, ¬(x.varmap[i]) ∨ ¬(y.varmap[j]) ∨ var.varmap[value])
end
end
end
return clauses
end
Base.:(==)(v::Var, w) = BinaryRelation{==}(v, w)
Base.:(<=)(v::Var, w) = BinaryRelation{<=}(v, w)
"Encode relation like x == 1"
function encode(rel::BinaryRelation{==, <:Var, <:Real})
x = rel.x
y = rel.y
if y ∉ domain(x)
error("$y is not in the domain of $x")
end
boolean = x.varmap[y]
return [boolean]
end
"Encode relation like x != 1"
function encode(rel::BinaryRelation{!=, <:Var, <:Real})
x = rel.x
y = rel.y
if y ∈ domain(x)
boolean = ¬(x.varmap[y])
end
return [boolean]
end
"Encode relation like x == y"
function encode(rel::BinaryRelation{==, <:Var, <:Var})
x = rel.x
y = rel.y
clauses = []
for i in domain(x)
if i in domain(y)
# (x == i) => (y == i)
push!(clauses, ¬(x.varmap[i]) ∨ (y.varmap[i]))
else
push!(clauses, ¬(x.varmap[i]))
end
end
for i in domain(y)
if i in domain(x)
# (y == i) => (x == i)
push!(clauses, ¬(y.varmap[i]) ∨ (x.varmap[i]))
else
push!(clauses, ¬(y.varmap[i]))
end
end
return clauses
end
function encode(rel::BinaryRelation{!=, <:Var, <:Var})
x = rel.x
y = rel.y
clauses = []
for i in domain(x)
if i in domain(y)
# (x == i) => (y != i)
push!(clauses, ¬(x.varmap[i]) ∨ ¬(y.varmap[i]))
end
end
return clauses
end
function parse_expression(varmap, ex)
op = operation(ex)
args = arguments(ex)
new_args = parse_expression.(Ref(varmap), args)
return op, new_args
end
parse_expression(varmap, ex::Term) = varmap[ex]
parse_expression(varmap, ex::Sym) = varmap[ex]
parse_expression(varmap, ex::Num) = parse_expression(varmap, value(ex))
parse_expression(varmap, ex::Real) = ex
"Parse a symbolic expression into a relation"
function parse_relation(varmap, ex)
op = operation(ex)
args = arguments(ex)
lhs = args[1]
rhs = args[2]
return BinaryRelation{op}(parse_expression(varmap, lhs), parse_expression(varmap, rhs))
end
# function parse_relation!(varmap, ex::Equation)
# varmap[ex.lhs] = parse_expression(varmap, ex.rhs)
# end
is_variable(ex) = !istree(ex) || (operation(ex) == getindex)
function process(constraint)
new_constraints = []
constraint2 = value(constraint)
op = operation(constraint2)
args = arguments(constraint2)
lhs = args[1]
rhs = args[2]
intermediates_generated = false
if !is_variable(lhs)
lhs = ReversePropagation.cse_equations(lhs)
append!(new_constraints, lhs[1])
lhs = lhs[2]
end
if !is_variable(rhs)
rhs = ReversePropagation.cse_equations(rhs)
append!(new_constraints, rhs[1])
rhs = rhs[2]
end
# @show new_constraints
# @show op, lhs, rhs
push!(new_constraints, op(lhs, rhs))
return new_constraints
end
process(constraints::Vector) = reduce(vcat, process(constraint) for constraint in constraints)
function parse_constraint!(domains, ex)
expr = value(ex)
op = operation(expr)
new_constraints = []
if op == ∈ # assumes right-hand side is an explicit set specifying the doomain
args = arguments(expr)
var = args[1]
domain = args[2]
domains[var] = domain
else
new_constraints = process(expr)
println()
# @show expr
# @show new_constraints
end
return domains, new_constraints
end
function parse_constraints(constraints)
# domains = Dict(var => -Inf..Inf for var in vars)
additional_vars = []
domains = Dict()
all_new_constraints = [] # excluding domain constraints
for constraint in constraints
# binarize constraints:
domains, new_constraints = parse_constraint!(domains, value(constraint))
for statement in new_constraints
if statement isa Assignment
push!(additional_vars, statement.lhs)
end
end
append!(all_new_constraints, new_constraints)
end
# append!(additional_vars, keys(domains))
return domains, all_new_constraints, additional_vars
end
Base.isless(x::Sym, y::Sym) = isless(x.name, y.name)
Base.isless(x::Term, y::Term) = isless(string(x), string(y))
Base.isless(x::Term, y::Sym) = isless(string(x), string(y))
Base.isless(x::Sym, y::Term) = isless(string(x), string(y))
struct ConstraintSatisfactionProblem
original_vars
additional_vars # from binarizing
domains
constraints
end
function ConstraintSatisfactionProblem(constraints)
domains, new_constraints, additional_vars = parse_constraints(constraints)
@show keys(domains)
vars = sort(identity.(keys(domains)))
additional_vars = sort(identity.(additional_vars))
return ConstraintSatisfactionProblem(vars, additional_vars, domains, new_constraints)
end
struct DiscreteCSP
original_vars
additional_vars
constraints
varmap # maps symbolic variables to the corresponding DiscreteVariable
end
DiscreteCSP(constraints) = DiscreteCSP(ConstraintSatisfactionProblem(constraints))
function DiscreteCSP(prob::ConstraintSatisfactionProblem)
variables = []
varmap = Dict()
new_constraints = []
for (var, domain) in prob.domains
variable = DiscreteVariable(var, domain)
push!(variables, variable)
push!(varmap, var => variable)
end
for constraint in prob.constraints
constraint = value(constraint)
if constraint isa Assignment
lhs = constraint.lhs
op, new_args = parse_expression(varmap, constraint.rhs) # makes a NodeVariable
# @show op, new_args
variable = NodeVariable(op, new_args[1], new_args[2], lhs)
push!(variables, variable)
push!(varmap, lhs => variable)
else
# @show constraint
push!(new_constraints, parse_relation(varmap, constraint))
end
end
original_vars = [varmap[var] for var in prob.original_vars]
additional_vars = [varmap[var] for var in prob.additional_vars]
return DiscreteCSP(original_vars, additional_vars, new_constraints, varmap)
end
function encode(prob::DiscreteCSP)
all_variables = []
all_clauses = []
variables = Any[prob.original_vars; prob.additional_vars]
domains = Dict(v.name => v.domain for v in variables)
for var in variables
append!(all_variables, var.booleans)
append!(all_clauses, clauses(var))
end
# @show prob.constraints
for constraint in prob.constraints
# @show constraint
append!(all_clauses, encode(constraint))
end
# @show all_variables
# @show all_clauses
return SymbolicSATProblem(identity.(all_variables), identity.(all_clauses))
end
function solve(prob::DiscreteCSP)
symbolic_sat_problem = encode(prob)
status, result_dict = solve(symbolic_sat_problem)
(status == :unsat) && return status, missing
# variables = Any[prob.original_vars; prob.additional_vars]
variables = prob.original_vars
return status, decode(prob, result_dict)
end
decode(prob::DiscreteCSP, result_dict) = Dict(v.name => decode(result_dict, v) for v in prob.original_vars)
function all_solutions(prob::DiscreteCSP)
sat_problem = encode(prob)
# variables = Any[prob.original_vars; prob.additional_vars]
variables = prob.original_vars
prob2 = encode(prob)
prob3 = prob2.p # SATProblem
sat_solutions = all_solutions(prob3)
if isempty(sat_solutions)
return sat_solutions
end
solutions = []
for solution in sat_solutions
push!(solutions, decode(prob, decode(prob2, solution)))
end
return solutions
end
| [
628,
628,
198,
198,
7249,
45755,
6892,
341,
90,
46,
11,
311,
11,
309,
92,
198,
220,
220,
220,
2124,
3712,
50,
198,
220,
220,
220,
331,
3712,
51,
220,
198,
437,
198,
198,
33,
3219,
6892,
341,
90,
46,
92,
7,
87,
3712,
55,
11,
331,
3712,
56,
8,
810,
1391,
46,
11,
55,
11,
56,
92,
796,
45755,
6892,
341,
90,
46,
11,
55,
11,
56,
92,
7,
87,
11,
331,
8,
628,
198,
87,
796,
8444,
8374,
43015,
7,
25,
87,
11,
352,
25,
20,
8,
198,
88,
796,
8444,
8374,
43015,
7,
25,
88,
11,
362,
25,
21,
8,
198,
198,
2,
45755,
6892,
341,
7,
855,
11,
2124,
11,
331,
8,
198,
2,
45755,
6892,
341,
7,
855,
11,
2124,
11,
352,
8,
198,
198,
2,
5765,
19081,
23907,
2977,
284,
2270,
510,
13934,
2316,
588,
2124,
1343,
331,
5299,
513,
290,
1059,
77,
560,
884,
355,
2124,
1343,
331,
19841,
1976,
198,
7249,
19081,
43015,
1279,
25,
12372,
198,
220,
220,
220,
1438,
198,
220,
220,
220,
1034,
220,
198,
220,
220,
220,
2124,
198,
220,
220,
220,
331,
198,
220,
220,
220,
7386,
198,
220,
220,
220,
1489,
2305,
504,
198,
220,
220,
220,
1401,
8899,
198,
437,
198,
198,
14881,
13,
12860,
7,
952,
3712,
9399,
11,
1401,
3712,
19667,
43015,
8,
796,
3601,
7,
952,
11,
17971,
7,
7785,
13,
3672,
8,
796,
29568,
7785,
13,
404,
5769,
3,
7,
7785,
13,
87,
828,
29568,
7785,
13,
88,
4008,
4943,
198,
198,
19796,
62,
27830,
7,
404,
11,
2124,
11,
331,
8,
796,
3297,
7,
33327,
7,
7248,
26933,
404,
7,
72,
11,
474,
8,
329,
1312,
287,
7386,
7,
87,
8,
329,
474,
287,
7386,
7,
88,
15437,
22305,
198,
198,
27830,
7,
85,
3712,
19852,
8,
796,
410,
13,
27830,
198,
2127,
2305,
504,
7,
85,
3712,
19852,
8,
796,
410,
13,
2127,
2305,
504,
198,
198,
27830,
7,
87,
3712,
15633,
8,
796,
2124,
25,
87,
198,
198,
1,
3237,
1489,
2305,
504,
2641,
281,
5408,
326,
1276,
307,
2087,
284,
262,
21004,
1,
198,
8344,
30753,
62,
2127,
2305,
504,
7,
85,
3712,
19667,
43015,
8,
796,
410,
13,
2127,
2305,
504,
18872,
103,
1489,
2305,
504,
7,
85,
13,
87,
8,
18872,
103,
1489,
2305,
504,
7,
85,
13,
88,
8,
198,
198,
8818,
19081,
43015,
7,
404,
11,
2124,
11,
331,
11,
1438,
28,
70,
641,
4948,
28955,
198,
220,
220,
220,
7386,
796,
1064,
62,
27830,
7,
404,
11,
2124,
11,
331,
8,
198,
220,
220,
220,
1489,
2305,
504,
796,
685,
43015,
7,
3672,
11,
1312,
8,
329,
1312,
18872,
230,
36525,
7,
27830,
15437,
198,
220,
220,
220,
1401,
8899,
796,
360,
713,
7,
72,
5218,
410,
329,
357,
72,
11,
410,
8,
287,
19974,
7,
27830,
11,
1489,
2305,
504,
4008,
198,
220,
220,
220,
19081,
43015,
7,
3672,
11,
1034,
11,
2124,
11,
331,
11,
7386,
11,
1489,
2305,
504,
11,
1401,
8899,
8,
198,
437,
628,
198,
198,
14881,
11207,
33747,
87,
3712,
19852,
11,
331,
8,
796,
19081,
43015,
7,
28200,
2124,
11,
331,
8,
198,
14881,
11207,
33747,
87,
11,
331,
3712,
19852,
8,
796,
19081,
43015,
7,
28200,
2124,
11,
331,
8,
198,
198,
14881,
11207,
9,
7,
87,
3712,
19852,
11,
331,
8,
796,
19081,
43015,
7,
25666,
2124,
11,
331,
8,
198,
14881,
11207,
9,
7,
87,
11,
331,
3712,
19852,
8,
796,
19081,
43015,
7,
25666,
2124,
11,
331,
8,
628,
198,
14881,
11207,
61,
7,
87,
3712,
19852,
11,
331,
8,
796,
19081,
43015,
7,
61,
11,
2124,
11,
331,
8,
198,
14881,
11207,
61,
7,
87,
11,
331,
3712,
19852,
8,
796,
19081,
43015,
7,
61,
11,
2124,
11,
331,
8,
628,
198,
8818,
31485,
7,
7785,
3712,
19667,
43015,
8,
628,
220,
220,
220,
1034,
796,
1401,
13,
404,
198,
220,
220,
220,
2124,
796,
1401,
13,
87,
220,
198,
220,
220,
220,
331,
796,
1401,
13,
88,
628,
220,
220,
220,
31485,
796,
3446,
62,
505,
7,
7785,
13,
2127,
2305,
504,
8,
628,
220,
220,
220,
1303,
1730,
351,
362,
1343,
1395,
198,
220,
220,
220,
611,
2124,
318,
64,
6416,
220,
198,
220,
220,
220,
220,
220,
220,
220,
329,
474,
287,
7386,
7,
7785,
13,
88,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1988,
796,
1034,
7,
87,
11,
474,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
331,
6624,
474,
5218,
1401,
6624,
1034,
7,
87,
11,
474,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
565,
64,
2664,
11,
1587,
105,
7,
88,
13,
85,
1670,
499,
58,
73,
12962,
18872,
101,
1401,
13,
85,
1670,
499,
58,
8367,
12962,
628,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
198,
220,
220,
220,
1303,
1730,
351,
2124,
1343,
362,
198,
220,
220,
220,
2073,
361,
331,
318,
64,
6416,
220,
198,
220,
220,
220,
220,
220,
220,
220,
329,
1312,
287,
7386,
7,
7785,
13,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1988,
796,
1034,
7,
72,
11,
331,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
2124,
6624,
1312,
5218,
1401,
6624,
1034,
7,
72,
11,
331,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
565,
64,
2664,
11,
1587,
105,
7,
87,
13,
85,
1670,
499,
58,
72,
12962,
18872,
101,
1401,
13,
85,
1670,
499,
58,
8367,
12962,
628,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
198,
220,
220,
220,
2073,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
6159,
2124,
4249,
331,
318,
1103,
220,
198,
220,
220,
220,
220,
220,
220,
220,
329,
1312,
287,
7386,
7,
7785,
13,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
329,
474,
287,
7386,
7,
7785,
13,
88,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1988,
796,
1034,
7,
72,
11,
474,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
2124,
6624,
1312,
11405,
331,
6624,
474,
5218,
1401,
6624,
1034,
7,
72,
11,
474,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
565,
64,
2664,
11,
1587,
105,
7,
87,
13,
85,
1670,
499,
58,
72,
12962,
18872,
101,
1587,
105,
7,
88,
13,
85,
1670,
499,
58,
73,
12962,
18872,
101,
1401,
13,
85,
1670,
499,
58,
8367,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
628,
220,
220,
220,
886,
628,
220,
220,
220,
1441,
31485,
198,
198,
437,
628,
628,
198,
198,
14881,
11207,
7,
855,
5769,
85,
3712,
19852,
11,
266,
8,
796,
45755,
6892,
341,
90,
855,
92,
7,
85,
11,
266,
8,
198,
14881,
11207,
7,
27,
28,
5769,
85,
3712,
19852,
11,
266,
8,
796,
45755,
6892,
341,
90,
27,
28,
92,
7,
85,
11,
266,
8,
628,
198,
1,
4834,
8189,
8695,
588,
2124,
6624,
352,
1,
198,
8818,
37773,
7,
2411,
3712,
33,
3219,
6892,
341,
90,
855,
11,
1279,
25,
19852,
11,
1279,
25,
15633,
30072,
628,
220,
220,
220,
2124,
796,
823,
13,
87,
220,
198,
220,
220,
220,
331,
796,
823,
13,
88,
628,
220,
220,
220,
611,
331,
18872,
231,
7386,
7,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
4049,
7203,
3,
88,
318,
407,
287,
262,
7386,
286,
720,
87,
4943,
198,
220,
220,
220,
886,
628,
220,
220,
220,
25131,
796,
2124,
13,
85,
1670,
499,
58,
88,
60,
628,
220,
220,
220,
1441,
685,
2127,
21052,
60,
198,
437,
198,
198,
1,
4834,
8189,
8695,
588,
2124,
14512,
352,
1,
198,
8818,
37773,
7,
2411,
3712,
33,
3219,
6892,
341,
90,
0,
28,
11,
1279,
25,
19852,
11,
1279,
25,
15633,
30072,
628,
220,
220,
220,
2124,
796,
823,
13,
87,
220,
198,
220,
220,
220,
331,
796,
823,
13,
88,
628,
220,
220,
220,
611,
331,
18872,
230,
7386,
7,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
25131,
796,
1587,
105,
7,
87,
13,
85,
1670,
499,
58,
88,
12962,
198,
220,
220,
220,
886,
628,
198,
220,
220,
220,
1441,
685,
2127,
21052,
60,
198,
437,
198,
198,
1,
4834,
8189,
8695,
588,
2124,
6624,
331,
1,
198,
8818,
37773,
7,
2411,
3712,
33,
3219,
6892,
341,
90,
855,
11,
1279,
25,
19852,
11,
1279,
25,
19852,
30072,
198,
220,
220,
220,
2124,
796,
823,
13,
87,
220,
198,
220,
220,
220,
331,
796,
823,
13,
88,
220,
628,
220,
220,
220,
31485,
796,
17635,
628,
220,
220,
220,
329,
1312,
287,
7386,
7,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
1312,
287,
7386,
7,
88,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
357,
87,
6624,
1312,
8,
5218,
357,
88,
6624,
1312,
8,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
565,
64,
2664,
11,
1587,
105,
7,
87,
13,
85,
1670,
499,
58,
72,
12962,
18872,
101,
357,
88,
13,
85,
1670,
499,
58,
72,
60,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
565,
64,
2664,
11,
1587,
105,
7,
87,
13,
85,
1670,
499,
58,
72,
60,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
886,
220,
198,
220,
220,
220,
886,
628,
220,
220,
220,
329,
1312,
287,
7386,
7,
88,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
1312,
287,
7386,
7,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
357,
88,
6624,
1312,
8,
5218,
357,
87,
6624,
1312,
8,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
565,
64,
2664,
11,
1587,
105,
7,
88,
13,
85,
1670,
499,
58,
72,
12962,
18872,
101,
357,
87,
13,
85,
1670,
499,
58,
72,
60,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
565,
64,
2664,
11,
1587,
105,
7,
88,
13,
85,
1670,
499,
58,
72,
60,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
198,
220,
220,
220,
1441,
31485,
220,
198,
437,
628,
198,
198,
8818,
37773,
7,
2411,
3712,
33,
3219,
6892,
341,
90,
0,
28,
11,
1279,
25,
19852,
11,
1279,
25,
19852,
30072,
198,
220,
220,
220,
2124,
796,
823,
13,
87,
220,
198,
220,
220,
220,
331,
796,
823,
13,
88,
220,
628,
220,
220,
220,
31485,
796,
17635,
628,
220,
220,
220,
329,
1312,
287,
7386,
7,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
1312,
287,
7386,
7,
88,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
357,
87,
6624,
1312,
8,
5218,
357,
88,
14512,
1312,
8,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
565,
64,
2664,
11,
1587,
105,
7,
87,
13,
85,
1670,
499,
58,
72,
12962,
18872,
101,
1587,
105,
7,
88,
13,
85,
1670,
499,
58,
72,
60,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
198,
220,
220,
220,
1441,
31485,
220,
198,
437,
628,
628,
198,
8818,
21136,
62,
38011,
7,
85,
1670,
499,
11,
409,
8,
220,
198,
220,
220,
220,
1034,
796,
4905,
7,
1069,
8,
198,
220,
220,
220,
26498,
796,
7159,
7,
1069,
8,
628,
220,
220,
220,
649,
62,
22046,
796,
21136,
62,
38011,
12195,
8134,
7,
85,
1670,
499,
828,
26498,
8,
628,
220,
220,
220,
1441,
1034,
11,
649,
62,
22046,
198,
437,
198,
198,
29572,
62,
38011,
7,
85,
1670,
499,
11,
409,
3712,
40596,
8,
796,
1401,
8899,
58,
1069,
60,
198,
29572,
62,
38011,
7,
85,
1670,
499,
11,
409,
3712,
43094,
8,
796,
1401,
8899,
58,
1069,
60,
198,
29572,
62,
38011,
7,
85,
1670,
499,
11,
409,
3712,
33111,
8,
796,
21136,
62,
38011,
7,
85,
1670,
499,
11,
1988,
7,
1069,
4008,
198,
29572,
62,
38011,
7,
85,
1670,
499,
11,
409,
3712,
15633,
8,
796,
409,
628,
198,
1,
10044,
325,
257,
18975,
5408,
656,
257,
8695,
1,
198,
8818,
21136,
62,
49501,
7,
85,
1670,
499,
11,
409,
8,
198,
220,
220,
220,
1034,
796,
4905,
7,
1069,
8,
198,
220,
220,
220,
26498,
796,
7159,
7,
1069,
8,
628,
220,
220,
220,
300,
11994,
796,
26498,
58,
16,
60,
198,
220,
220,
220,
9529,
82,
796,
26498,
58,
17,
60,
628,
220,
220,
220,
198,
220,
220,
220,
1441,
45755,
6892,
341,
90,
404,
92,
7,
29572,
62,
38011,
7,
85,
1670,
499,
11,
300,
11994,
828,
21136,
62,
38011,
7,
85,
1670,
499,
11,
9529,
82,
4008,
198,
198,
437,
628,
198,
2,
2163,
21136,
62,
49501,
0,
7,
85,
1670,
499,
11,
409,
3712,
23588,
341,
8,
198,
220,
220,
220,
220,
198,
2,
220,
220,
220,
220,
1401,
8899,
58,
1069,
13,
75,
11994,
60,
796,
21136,
62,
38011,
7,
85,
1670,
499,
11,
409,
13,
81,
11994,
8,
220,
220,
220,
198,
198,
2,
886,
198,
198,
271,
62,
45286,
7,
1069,
8,
796,
5145,
396,
631,
7,
1069,
8,
8614,
357,
27184,
7,
1069,
8,
6624,
651,
9630,
8,
198,
198,
8818,
1429,
7,
1102,
2536,
2913,
8,
628,
220,
220,
220,
649,
62,
1102,
2536,
6003,
796,
17635,
628,
220,
220,
220,
32315,
17,
796,
1988,
7,
1102,
2536,
2913,
8,
628,
220,
220,
220,
1034,
796,
4905,
7,
1102,
2536,
2913,
17,
8,
198,
220,
220,
220,
26498,
796,
7159,
7,
1102,
2536,
2913,
17,
8,
628,
220,
220,
220,
300,
11994,
796,
26498,
58,
16,
60,
198,
220,
220,
220,
9529,
82,
796,
26498,
58,
17,
60,
628,
220,
220,
220,
30127,
689,
62,
27568,
796,
3991,
628,
220,
220,
220,
611,
5145,
271,
62,
45286,
7,
75,
11994,
8,
198,
220,
220,
220,
220,
220,
220,
220,
300,
11994,
796,
31849,
24331,
363,
341,
13,
66,
325,
62,
4853,
602,
7,
75,
11994,
8,
628,
220,
220,
220,
220,
220,
220,
220,
24443,
0,
7,
3605,
62,
1102,
2536,
6003,
11,
300,
11994,
58,
16,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
300,
11994,
796,
300,
11994,
58,
17,
60,
628,
220,
220,
220,
886,
628,
220,
220,
220,
611,
5145,
271,
62,
45286,
7,
81,
11994,
8,
198,
220,
220,
220,
220,
220,
220,
220,
9529,
82,
796,
31849,
24331,
363,
341,
13,
66,
325,
62,
4853,
602,
7,
81,
11994,
8,
628,
220,
220,
220,
220,
220,
220,
220,
24443,
0,
7,
3605,
62,
1102,
2536,
6003,
11,
9529,
82,
58,
16,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
9529,
82,
796,
9529,
82,
58,
17,
60,
628,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
2488,
12860,
649,
62,
1102,
2536,
6003,
198,
220,
220,
220,
1303,
2488,
12860,
1034,
11,
300,
11994,
11,
9529,
82,
198,
220,
220,
220,
4574,
0,
7,
3605,
62,
1102,
2536,
6003,
11,
1034,
7,
75,
11994,
11,
9529,
82,
4008,
628,
220,
220,
220,
1441,
649,
62,
1102,
2536,
6003,
198,
198,
437,
628,
198,
14681,
7,
1102,
2536,
6003,
3712,
38469,
8,
796,
4646,
7,
85,
9246,
11,
1429,
7,
1102,
2536,
2913,
8,
329,
32315,
287,
17778,
8,
198,
220,
220,
220,
220,
628,
198,
198,
8818,
21136,
62,
1102,
2536,
2913,
0,
7,
3438,
1299,
11,
409,
8,
628,
220,
220,
220,
44052,
796,
1988,
7,
1069,
8,
198,
220,
220,
220,
1034,
796,
4905,
7,
31937,
8,
628,
220,
220,
220,
649,
62,
1102,
2536,
6003,
796,
17635,
628,
220,
220,
220,
611,
1034,
6624,
18872,
230,
220,
220,
1303,
18533,
826,
12,
4993,
1735,
318,
281,
7952,
900,
31577,
262,
27666,
391,
628,
220,
220,
220,
220,
220,
220,
220,
26498,
796,
7159,
7,
31937,
8,
628,
220,
220,
220,
220,
220,
220,
220,
1401,
796,
26498,
58,
16,
60,
198,
220,
220,
220,
220,
220,
220,
220,
7386,
796,
26498,
58,
17,
60,
628,
220,
220,
220,
220,
220,
220,
220,
18209,
58,
7785,
60,
796,
7386,
628,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
649,
62,
1102,
2536,
6003,
796,
1429,
7,
31937,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
44872,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
2488,
12860,
44052,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
2488,
12860,
649,
62,
1102,
2536,
6003,
628,
220,
220,
220,
886,
628,
220,
220,
220,
1441,
18209,
11,
649,
62,
1102,
2536,
6003,
198,
198,
437,
628,
198,
8818,
21136,
62,
1102,
2536,
6003,
7,
1102,
2536,
6003,
8,
198,
220,
220,
220,
1303,
18209,
796,
360,
713,
7,
7785,
5218,
532,
18943,
492,
18943,
329,
1401,
287,
410,
945,
8,
628,
220,
220,
220,
3224,
62,
85,
945,
796,
17635,
198,
220,
220,
220,
18209,
796,
360,
713,
3419,
198,
220,
220,
220,
477,
62,
3605,
62,
1102,
2536,
6003,
796,
17635,
220,
1303,
23494,
7386,
17778,
628,
220,
220,
220,
329,
32315,
287,
17778,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
9874,
283,
1096,
17778,
25,
220,
198,
220,
220,
220,
220,
220,
220,
220,
18209,
11,
649,
62,
1102,
2536,
6003,
796,
21136,
62,
1102,
2536,
2913,
0,
7,
3438,
1299,
11,
1988,
7,
1102,
2536,
2913,
4008,
628,
220,
220,
220,
220,
220,
220,
220,
329,
2643,
287,
649,
62,
1102,
2536,
6003,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
2643,
318,
64,
50144,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
2860,
1859,
62,
85,
945,
11,
2643,
13,
75,
11994,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
628,
220,
220,
220,
220,
220,
220,
220,
24443,
0,
7,
439,
62,
3605,
62,
1102,
2536,
6003,
11,
649,
62,
1102,
2536,
6003,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
24443,
0,
7,
2860,
1859,
62,
85,
945,
11,
8251,
7,
3438,
1299,
4008,
628,
220,
220,
220,
1441,
18209,
11,
477,
62,
3605,
62,
1102,
2536,
6003,
11,
3224,
62,
85,
945,
198,
437,
628,
628,
198,
14881,
13,
271,
1203,
7,
87,
3712,
43094,
11,
331,
3712,
43094,
8,
796,
318,
1203,
7,
87,
13,
3672,
11,
331,
13,
3672,
8,
198,
14881,
13,
271,
1203,
7,
87,
3712,
40596,
11,
331,
3712,
40596,
8,
796,
318,
1203,
7,
8841,
7,
87,
828,
4731,
7,
88,
4008,
198,
14881,
13,
271,
1203,
7,
87,
3712,
40596,
11,
331,
3712,
43094,
8,
796,
318,
1203,
7,
8841,
7,
87,
828,
4731,
7,
88,
4008,
198,
14881,
13,
271,
1203,
7,
87,
3712,
43094,
11,
331,
3712,
40596,
8,
796,
318,
1203,
7,
8841,
7,
87,
828,
4731,
7,
88,
4008,
198,
198,
7249,
1482,
2536,
2913,
50,
17403,
2673,
40781,
220,
198,
220,
220,
220,
2656,
62,
85,
945,
220,
198,
220,
220,
220,
3224,
62,
85,
945,
220,
220,
1303,
422,
9874,
283,
2890,
198,
220,
220,
220,
18209,
198,
220,
220,
220,
17778,
220,
198,
437,
198,
198,
8818,
1482,
2536,
2913,
50,
17403,
2673,
40781,
7,
1102,
2536,
6003,
8,
628,
220,
220,
220,
18209,
11,
649,
62,
1102,
2536,
6003,
11,
3224,
62,
85,
945,
796,
21136,
62,
1102,
2536,
6003,
7,
1102,
2536,
6003,
8,
198,
220,
220,
220,
2488,
12860,
8251,
7,
3438,
1299,
8,
198,
220,
220,
220,
410,
945,
796,
3297,
7,
738,
414,
12195,
13083,
7,
3438,
1299,
22305,
198,
220,
220,
220,
3224,
62,
85,
945,
796,
3297,
7,
738,
414,
12195,
2860,
1859,
62,
85,
945,
4008,
628,
220,
220,
220,
1441,
1482,
2536,
2913,
50,
17403,
2673,
40781,
7,
85,
945,
11,
3224,
62,
85,
945,
11,
18209,
11,
649,
62,
1102,
2536,
6003,
8,
198,
437,
628,
198,
7249,
8444,
8374,
34,
4303,
198,
220,
220,
220,
2656,
62,
85,
945,
198,
220,
220,
220,
3224,
62,
85,
945,
198,
220,
220,
220,
17778,
198,
220,
220,
220,
1401,
8899,
220,
220,
1303,
8739,
18975,
9633,
284,
262,
11188,
8444,
8374,
43015,
198,
437,
628,
198,
15642,
8374,
34,
4303,
7,
1102,
2536,
6003,
8,
796,
8444,
8374,
34,
4303,
7,
3103,
2536,
2913,
50,
17403,
2673,
40781,
7,
1102,
2536,
6003,
4008,
198,
198,
8818,
8444,
8374,
34,
4303,
7,
1676,
65,
3712,
3103,
2536,
2913,
50,
17403,
2673,
40781,
8,
198,
220,
220,
220,
9633,
796,
17635,
198,
220,
220,
220,
1401,
8899,
796,
360,
713,
3419,
628,
220,
220,
220,
649,
62,
1102,
2536,
6003,
796,
17635,
628,
220,
220,
220,
329,
357,
7785,
11,
7386,
8,
287,
1861,
13,
3438,
1299,
198,
220,
220,
220,
220,
220,
220,
220,
7885,
796,
8444,
8374,
43015,
7,
7785,
11,
7386,
8,
198,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
25641,
2977,
11,
7885,
8,
198,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
85,
1670,
499,
11,
1401,
5218,
7885,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
329,
32315,
287,
1861,
13,
1102,
2536,
6003,
220,
198,
220,
220,
220,
220,
220,
220,
220,
32315,
796,
1988,
7,
1102,
2536,
2913,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
32315,
318,
64,
50144,
220,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
300,
11994,
796,
32315,
13,
75,
11994,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1034,
11,
649,
62,
22046,
796,
21136,
62,
38011,
7,
85,
1670,
499,
11,
32315,
13,
81,
11994,
8,
220,
220,
1303,
1838,
257,
19081,
43015,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
2488,
12860,
1034,
11,
649,
62,
22046,
220,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7885,
796,
19081,
43015,
7,
404,
11,
649,
62,
22046,
58,
16,
4357,
649,
62,
22046,
58,
17,
4357,
300,
11994,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
25641,
2977,
11,
7885,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
85,
1670,
499,
11,
300,
11994,
5218,
7885,
8,
628,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
2488,
12860,
32315,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
3605,
62,
1102,
2536,
6003,
11,
21136,
62,
49501,
7,
85,
1670,
499,
11,
32315,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2656,
62,
85,
945,
796,
685,
85,
1670,
499,
58,
7785,
60,
329,
1401,
287,
1861,
13,
14986,
62,
85,
945,
60,
198,
220,
220,
220,
3224,
62,
85,
945,
796,
685,
85,
1670,
499,
58,
7785,
60,
329,
1401,
287,
1861,
13,
2860,
1859,
62,
85,
945,
60,
198,
220,
220,
220,
198,
220,
220,
220,
1441,
8444,
8374,
34,
4303,
7,
14986,
62,
85,
945,
11,
3224,
62,
85,
945,
11,
649,
62,
1102,
2536,
6003,
11,
1401,
8899,
8,
198,
437,
628,
628,
198,
8818,
37773,
7,
1676,
65,
3712,
15642,
8374,
34,
4303,
8,
198,
220,
220,
220,
477,
62,
25641,
2977,
796,
17635,
198,
220,
220,
220,
477,
62,
565,
64,
2664,
796,
17635,
628,
220,
220,
220,
9633,
796,
4377,
58,
1676,
65,
13,
14986,
62,
85,
945,
26,
1861,
13,
2860,
1859,
62,
85,
945,
60,
198,
220,
220,
220,
220,
628,
220,
220,
220,
18209,
796,
360,
713,
7,
85,
13,
3672,
5218,
410,
13,
27830,
329,
410,
287,
9633,
8,
628,
220,
220,
220,
329,
1401,
287,
9633,
198,
220,
220,
220,
220,
220,
220,
220,
24443,
0,
7,
439,
62,
25641,
2977,
11,
1401,
13,
2127,
2305,
504,
8,
198,
220,
220,
220,
220,
220,
220,
220,
24443,
0,
7,
439,
62,
565,
64,
2664,
11,
31485,
7,
7785,
4008,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
2488,
12860,
1861,
13,
1102,
2536,
6003,
628,
220,
220,
220,
329,
32315,
287,
1861,
13,
1102,
2536,
6003,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
2488,
12860,
32315,
198,
220,
220,
220,
220,
220,
220,
220,
24443,
0,
7,
439,
62,
565,
64,
2664,
11,
37773,
7,
1102,
2536,
2913,
4008,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
2488,
12860,
477,
62,
25641,
2977,
220,
198,
220,
220,
220,
1303,
2488,
12860,
477,
62,
565,
64,
2664,
220,
628,
220,
220,
220,
1441,
41327,
4160,
50,
1404,
40781,
7,
738,
414,
12195,
439,
62,
25641,
2977,
828,
5369,
12195,
439,
62,
565,
64,
2664,
4008,
198,
437,
628,
198,
8818,
8494,
7,
1676,
65,
3712,
15642,
8374,
34,
4303,
8,
198,
220,
220,
220,
18975,
62,
49720,
62,
45573,
796,
37773,
7,
1676,
65,
8,
628,
220,
220,
220,
3722,
11,
1255,
62,
11600,
796,
8494,
7,
1837,
2022,
4160,
62,
49720,
62,
45573,
8,
628,
220,
220,
220,
357,
13376,
6624,
1058,
13271,
265,
8,
11405,
1441,
3722,
11,
4814,
628,
220,
220,
220,
1303,
9633,
796,
4377,
58,
1676,
65,
13,
14986,
62,
85,
945,
26,
1861,
13,
2860,
1859,
62,
85,
945,
60,
198,
220,
220,
220,
9633,
796,
1861,
13,
14986,
62,
85,
945,
628,
220,
220,
220,
1441,
3722,
11,
36899,
7,
1676,
65,
11,
1255,
62,
11600,
8,
198,
437,
198,
198,
12501,
1098,
7,
1676,
65,
3712,
15642,
8374,
34,
4303,
11,
1255,
62,
11600,
8,
796,
360,
713,
7,
85,
13,
3672,
5218,
36899,
7,
20274,
62,
11600,
11,
410,
8,
329,
410,
287,
1861,
13,
14986,
62,
85,
945,
8,
198,
220,
220,
220,
220,
628,
198,
8818,
477,
62,
82,
14191,
7,
1676,
65,
3712,
15642,
8374,
34,
4303,
8,
198,
220,
220,
220,
3332,
62,
45573,
796,
37773,
7,
1676,
65,
8,
198,
220,
220,
220,
1303,
9633,
796,
4377,
58,
1676,
65,
13,
14986,
62,
85,
945,
26,
1861,
13,
2860,
1859,
62,
85,
945,
60,
198,
220,
220,
220,
9633,
796,
1861,
13,
14986,
62,
85,
945,
628,
220,
220,
220,
1861,
17,
796,
37773,
7,
1676,
65,
8,
198,
220,
220,
220,
1861,
18,
796,
1861,
17,
13,
79,
220,
220,
1303,
29020,
40781,
628,
220,
220,
220,
3332,
62,
82,
14191,
796,
477,
62,
82,
14191,
7,
1676,
65,
18,
8,
628,
220,
220,
220,
611,
318,
28920,
7,
49720,
62,
82,
14191,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
3332,
62,
82,
14191,
220,
198,
220,
220,
220,
886,
628,
220,
220,
220,
8136,
796,
17635,
628,
220,
220,
220,
329,
4610,
287,
3332,
62,
82,
14191,
198,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
82,
14191,
11,
36899,
7,
1676,
65,
11,
36899,
7,
1676,
65,
17,
11,
4610,
22305,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1441,
8136,
198,
198,
437,
198
] | 2.205711 | 4,623 |
using Test
using FdeSolver
@testset "FdeSolver.jl" begin
b = FdeSolver.greet()
@test @isdefined(b)
end
| [
3500,
6208,
198,
3500,
376,
2934,
50,
14375,
198,
198,
31,
9288,
2617,
366,
37,
2934,
50,
14375,
13,
20362,
1,
2221,
198,
220,
220,
220,
275,
796,
376,
2934,
50,
14375,
13,
70,
2871,
3419,
198,
220,
220,
220,
2488,
9288,
2488,
271,
23211,
7,
65,
8,
198,
437,
198
] | 2.196078 | 51 |
struct Characteristic <: AbstractCharacteristic
ws::WeightSpec
Fξ::Symbol
X::Dict{Symbol, Symbol}
end
#builds a characteristic object, which includes cross-sectional weights and
#weight coefficients
function Characteristic(ws::WeightSpec, Fξ::Symbol)
Fw = Symbol(:w, ws.weightprefix, Fξ)
#a couple of helper funtions for consistent renaming
lagname(s::Symbol) = Symbol(:L, s)
retname(s::Symbol) = Symbol(:R, s)
rettname(s::Symbol) = Symbol(:Rt, s)
#WARNING: HARDCODE ALERT!!!
X = Dict{Symbol, Symbol}()
X[:Fw] = Fw
X[:FLw] = lagname(X[:Fw])
X[:FLLw] = lagname(X[:FLw])
X[:FLLLw] = lagname(X[:FLLw])
#not used for now
X[:FRLw] = retname(X[:FLw])
X[:FRLLw] = retname(X[:FLLw])
X[:FRtw] = rettname(X[:Fw])
return Characteristic(ws, Fξ, X)
end
weightfields(ξ::Characteristic) = collect(values(ξ.X))
Characteristic(ξ::Characteristic, Fξ::Symbol, X::Dict
) = Characteristic(ξ.ws, Fξ, X)
demeanξ(ξraw::AbstractVector{<:Union{Real, Missing}}) = ξraw .- mean(skipmissing(ξraw))
#this gives the quantile fo each value in an array
#would have preferred the ecdf function, but it doesn't play nice with
#containers that can hold missing values, even if none are actually held
#=function quantiles(ξraw::AbstractVector{<:Union{Real,Missing}})
(length(ξraw) .- competerank(ξraw,rev=true) .+ 1)./length(ξraw)
end=#
function quantiles(ξraw::AbstractVector{<:Union{Real,Missing}})
(length(ξraw) .- competerank(ξraw,rev=true) .+ 1)./length(ξraw)
end
#alternate procedure
#=function quantiles(ξraw::AbstractVector{<:Union{Real,Missing}})
F = ecdf(ξraw |> Vector{Float64})
return map(F, ξraw)
end=#
#conditions each slice of the panel for a particular characteristic
function conditionξ!(F::Function, panel::AbstractDataFrame, Fξraw::Symbol, Fξ::Symbol)::Nothing
(sum((!ismissing).(panel[!, Fξ])) > 0) && error("$Fξ in panel already contains data")
spanels::GroupedDataFrame = groupby(view(panel, (!ismissing).(panel[!,Fξraw]), :), :date)
Threads.@threads for i ∈ 1:length(spanels)
spanel::SubDataFrame = spanels[i]
Fξsubcol::SubArray = spanel[!, Fξ]
Fξsubcol .= F(spanel[!, Fξraw])
end
return nothing
end
#=standard conditioning of a characteristic column
function conditionξ!(panel::AbstractDataFrame, Fξraw::Symbol;
prefix::Symbol = PARAM[:charprefix],
Fξ::Symbol = Symbol(prefix, Fξraw))::Nothing
#allocate the data
ξtype::Type = Union{eltype(panel[!,Fξraw]), Missing}
panel[!, Fξ] = missings(ξtype, size(panel,1))
conditionξ!(demeanξ, panel, Fξraw, Fξ)
return nothing
end
#apply a quantile transformation before demeaning
function conditionξquantile!(panel::AbstractDataFrame, Fξraw::Symbol;
prefix::Symbol = PARAM[:charprefix],
Fξ::Symbol = Symbol(prefix, Fξraw))::Nothing
#allocate the data
panel[!, Fξ] = Vector{Union{eltype(panel[!,Fξraw])}}(undef, size(panel,1))
conditionξ!(demeanξ ∘ quantiles, panel, Fξraw, Fξ)
return nothing
end=#
| [
198,
7249,
15684,
2569,
1279,
25,
27741,
27275,
2569,
198,
220,
266,
82,
3712,
25844,
22882,
198,
220,
376,
138,
122,
3712,
13940,
23650,
198,
220,
1395,
3712,
35,
713,
90,
13940,
23650,
11,
38357,
92,
198,
437,
628,
198,
2,
11249,
82,
257,
16704,
2134,
11,
543,
3407,
3272,
12,
44330,
19590,
290,
198,
2,
6551,
44036,
198,
8818,
15684,
2569,
7,
18504,
3712,
25844,
22882,
11,
376,
138,
122,
3712,
13940,
23650,
8,
628,
220,
376,
86,
796,
38357,
7,
25,
86,
11,
266,
82,
13,
6551,
40290,
11,
376,
138,
122,
8,
628,
220,
1303,
64,
3155,
286,
31904,
1257,
45240,
329,
6414,
8851,
3723,
198,
220,
19470,
3672,
7,
82,
3712,
13940,
23650,
8,
796,
38357,
7,
25,
43,
11,
264,
8,
198,
220,
1005,
3672,
7,
82,
3712,
13940,
23650,
8,
796,
38357,
7,
25,
49,
11,
264,
8,
198,
220,
302,
926,
3672,
7,
82,
3712,
13940,
23650,
8,
796,
38357,
7,
25,
49,
83,
11,
264,
8,
628,
220,
1303,
31502,
25,
43638,
9697,
16820,
8355,
17395,
10185,
198,
220,
1395,
796,
360,
713,
90,
13940,
23650,
11,
38357,
92,
3419,
198,
220,
1395,
58,
25,
37,
86,
60,
796,
376,
86,
198,
220,
1395,
58,
25,
3697,
86,
60,
796,
19470,
3672,
7,
55,
58,
25,
37,
86,
12962,
198,
220,
1395,
58,
25,
37,
3069,
86,
60,
796,
19470,
3672,
7,
55,
58,
25,
3697,
86,
12962,
198,
220,
1395,
58,
25,
37,
3069,
43,
86,
60,
796,
19470,
3672,
7,
55,
58,
25,
37,
3069,
86,
12962,
198,
220,
1303,
1662,
973,
329,
783,
198,
220,
1395,
58,
25,
37,
7836,
86,
60,
796,
1005,
3672,
7,
55,
58,
25,
3697,
86,
12962,
198,
220,
1395,
58,
25,
10913,
3069,
86,
60,
796,
1005,
3672,
7,
55,
58,
25,
37,
3069,
86,
12962,
628,
220,
1395,
58,
25,
10913,
4246,
60,
796,
302,
926,
3672,
7,
55,
58,
25,
37,
86,
12962,
198,
220,
1441,
15684,
2569,
7,
18504,
11,
376,
138,
122,
11,
1395,
8,
198,
437,
198,
198,
6551,
25747,
7,
138,
122,
3712,
27275,
2569,
8,
796,
2824,
7,
27160,
7,
138,
122,
13,
55,
4008,
198,
27275,
2569,
7,
138,
122,
3712,
27275,
2569,
11,
220,
376,
138,
122,
3712,
13940,
23650,
11,
1395,
3712,
35,
713,
198,
220,
1267,
796,
15684,
2569,
7,
138,
122,
13,
18504,
11,
376,
138,
122,
11,
1395,
8,
198,
198,
9536,
11025,
138,
122,
7,
138,
122,
1831,
3712,
23839,
38469,
90,
27,
25,
38176,
90,
15633,
11,
25639,
11709,
8,
796,
7377,
122,
1831,
764,
12,
1612,
7,
48267,
45688,
7,
138,
122,
1831,
4008,
198,
2,
5661,
3607,
262,
5554,
576,
11511,
1123,
1988,
287,
281,
7177,
198,
2,
19188,
423,
9871,
262,
9940,
7568,
2163,
11,
475,
340,
1595,
470,
711,
3621,
351,
198,
2,
3642,
50221,
326,
460,
1745,
4814,
3815,
11,
772,
611,
4844,
389,
1682,
2714,
198,
2,
28,
8818,
5554,
2915,
7,
138,
122,
1831,
3712,
23839,
38469,
90,
27,
25,
38176,
90,
15633,
11,
43730,
11709,
8,
198,
220,
357,
13664,
7,
138,
122,
1831,
8,
764,
12,
2307,
263,
962,
7,
138,
122,
1831,
11,
18218,
28,
7942,
8,
764,
10,
352,
737,
14,
13664,
7,
138,
122,
1831,
8,
198,
437,
46249,
198,
198,
8818,
5554,
2915,
7,
138,
122,
1831,
3712,
23839,
38469,
90,
27,
25,
38176,
90,
15633,
11,
43730,
11709,
8,
198,
220,
357,
13664,
7,
138,
122,
1831,
8,
764,
12,
2307,
263,
962,
7,
138,
122,
1831,
11,
18218,
28,
7942,
8,
764,
10,
352,
737,
14,
13664,
7,
138,
122,
1831,
8,
198,
437,
198,
198,
2,
33645,
378,
8771,
198,
2,
28,
8818,
5554,
2915,
7,
138,
122,
1831,
3712,
23839,
38469,
90,
27,
25,
38176,
90,
15633,
11,
43730,
11709,
8,
198,
220,
376,
796,
9940,
7568,
7,
138,
122,
1831,
930,
29,
20650,
90,
43879,
2414,
30072,
198,
220,
1441,
3975,
7,
37,
11,
7377,
122,
1831,
8,
198,
437,
46249,
628,
198,
2,
17561,
1756,
1123,
16416,
286,
262,
6103,
329,
257,
1948,
16704,
198,
8818,
4006,
138,
122,
0,
7,
37,
3712,
22203,
11,
6103,
3712,
23839,
6601,
19778,
11,
376,
138,
122,
1831,
3712,
13940,
23650,
11,
376,
138,
122,
3712,
13940,
23650,
2599,
25,
18465,
628,
220,
357,
16345,
19510,
0,
1042,
747,
278,
737,
7,
35330,
58,
28265,
376,
138,
122,
60,
4008,
1875,
657,
8,
11405,
4049,
7203,
3,
37,
138,
122,
287,
6103,
1541,
4909,
1366,
4943,
198,
220,
11506,
1424,
3712,
13247,
276,
6601,
19778,
796,
1448,
1525,
7,
1177,
7,
35330,
11,
22759,
1042,
747,
278,
737,
7,
35330,
58,
28265,
37,
138,
122,
1831,
46570,
1058,
828,
1058,
4475,
8,
198,
220,
14122,
82,
13,
31,
16663,
82,
329,
1312,
18872,
230,
352,
25,
13664,
7,
12626,
1424,
8,
198,
220,
220,
220,
220,
220,
11506,
417,
3712,
7004,
6601,
19778,
796,
11506,
1424,
58,
72,
60,
198,
220,
220,
220,
220,
220,
376,
138,
122,
7266,
4033,
3712,
7004,
19182,
796,
11506,
417,
58,
28265,
376,
138,
122,
60,
198,
220,
220,
220,
220,
220,
376,
138,
122,
7266,
4033,
764,
28,
376,
7,
12626,
417,
58,
28265,
376,
138,
122,
1831,
12962,
198,
220,
886,
628,
220,
1441,
2147,
198,
437,
198,
198,
2,
28,
20307,
21143,
286,
257,
16704,
5721,
198,
8818,
4006,
138,
122,
0,
7,
35330,
3712,
23839,
6601,
19778,
11,
376,
138,
122,
1831,
3712,
13940,
23650,
26,
198,
220,
21231,
3712,
13940,
23650,
796,
29463,
2390,
58,
25,
10641,
40290,
4357,
198,
220,
376,
138,
122,
3712,
13940,
23650,
796,
38357,
7,
40290,
11,
376,
138,
122,
1831,
8,
2599,
25,
18465,
628,
220,
1303,
439,
13369,
262,
1366,
198,
220,
7377,
122,
4906,
3712,
6030,
796,
4479,
90,
417,
4906,
7,
35330,
58,
28265,
37,
138,
122,
1831,
46570,
25639,
92,
198,
220,
6103,
58,
28265,
376,
138,
122,
60,
796,
2051,
654,
7,
138,
122,
4906,
11,
2546,
7,
35330,
11,
16,
4008,
628,
220,
4006,
138,
122,
0,
7,
9536,
11025,
138,
122,
11,
6103,
11,
376,
138,
122,
1831,
11,
376,
138,
122,
8,
198,
220,
1441,
2147,
198,
437,
198,
198,
2,
39014,
257,
5554,
576,
13389,
878,
1357,
68,
7574,
198,
8818,
4006,
138,
122,
40972,
576,
0,
7,
35330,
3712,
23839,
6601,
19778,
11,
376,
138,
122,
1831,
3712,
13940,
23650,
26,
198,
220,
21231,
3712,
13940,
23650,
796,
29463,
2390,
58,
25,
10641,
40290,
4357,
198,
220,
376,
138,
122,
3712,
13940,
23650,
796,
38357,
7,
40290,
11,
376,
138,
122,
1831,
8,
2599,
25,
18465,
628,
220,
1303,
439,
13369,
262,
1366,
198,
220,
6103,
58,
28265,
376,
138,
122,
60,
796,
20650,
90,
38176,
90,
417,
4906,
7,
35330,
58,
28265,
37,
138,
122,
1831,
12962,
11709,
7,
917,
891,
11,
2546,
7,
35330,
11,
16,
4008,
628,
220,
4006,
138,
122,
0,
7,
9536,
11025,
138,
122,
18872,
246,
5554,
2915,
11,
6103,
11,
376,
138,
122,
1831,
11,
376,
138,
122,
8,
198,
220,
1441,
2147,
198,
437,
46249,
198
] | 2.522669 | 1,169 |
# licor_split.jl
#
# Split Li-7200 TXT files into GHG archives
#
# Jeremy Rüffer
# Thünen Institut
# Institut für Agrarklimaschutz
# Junior Research Group NITROSPHERE
# Julia 0.7
# 20.05.2014
# Last Edit: 12.04.2019
"# licor_split(source::String,destination::String;verbose::Bool=false)
Split an Li-7200 text file into small files in the standard GHG format. If possible, files will start on the usual four hour marks (0000, 0400, 0800, etc.).
`licor_split(source,destination)` Split Li7200 files in the source directors\n
* **source**::String = Directory of Li-7200 text files with names similar to 2016-03-17T104500.txt
* **destination**::String = Destination directory\n\n
---\n
#### Keywords:\n
* verbose::Bool = Display information as the function runs, TRUE is default
\n\n"
function licor_split(Dr::String,Dest::String;verbose::Bool=true)
###################
## Definitions ##
###################
# Dr = Source Directory
# Dest = Destination Directory
####################
## Parse Inputs ##
####################
# Check Directory Validity
if ~isdir(Dr) | ~isdir(Dest)
error("Source or destination directory does not exist")
end
(files,folders) = dirlist(Dr,regex=r"\d{4}-\d{2}-\d{2}T\d{6}\.txt$",recur=1) # List TXT files
#####################
## Process Files ##
#####################
if isempty(files)
println("No Metdata files to split")
else
if verbose
println("=== Splitting Metdata Files ===")
end
end
time = DateTime[]
starttime = DateTime[]
endtime = DateTime[]
fname = String[]
header = Array{String}(undef,0) # Initialized header array
sentinel = 20; # Sentinel value for header while loop
for i=1:1:length(files)
if verbose
println(" " * files[i])
end
s = stat(files[i]) # Get file info
fid = open(files[i],"r")
# Load Header
j = 1;
l = "Bierchen" # Needs any string at least 5 characters long
while l[1:5] != "DATAH" && j < sentinel
l = readline(fid,keep=true)
header = [header;l]
j += 1
end
data_pos = position(fid) # Position of where the data starts
#Get Start Time
l = readline(fid,keep=true)
ft = findall(x -> x == '\t',l)
starttime = DateTime(l[ft[6]+1:ft[8]-1],"yyyy-mm-dd\tHH:MM:SS:sss")
# Get End Time
endline = true
lastpos = 4 # Characters from the end of the file where the last line begins
while endline == true
seek(fid,s.size-lastpos)
l = readline(fid,keep=true)
if eof(fid) == false
endline = false
else
lastpos += 2
end
end
l = readline(fid,keep=true)
ft = findall(x -> x == '\t',l)
endtime = DateTime(l[ft[6]+1:ft[8]-1],"yyyy-mm-dd\tHH:MM:SS:sss")
# Split the File
seek(fid,data_pos) # Move the cursor to the start of the data
fid2 = open(files[i]);close(fid2)
next_start = []
first_file = true
while eof(fid) == false
if isopen(fid2) == false
# No output stream available
# Open new output file from previous line
if first_file == true
# There is not previously loaded line, load one now
l = readline(fid,keep=true)
first_file = false
end
# Calculate last value of file
ft = findall(x -> x == '\t',l)
temp_start = DateTime(l[ft[6]+1:ft[8]-1],"yyyy-mm-dd\tHH:MM:SS:sss")
HH2 = Dates.Hour[]
DD2 = Dates.Day(temp_start)
if Dates.Hour(0) <= Dates.Hour(temp_start) < Dates.Hour(4)
HH2 = Dates.Hour(4)
elseif Dates.Hour(4) <= Dates.Hour(temp_start) < Dates.Hour(8)
HH2 = Dates.Hour(8)
elseif Dates.Hour(8) <= Dates.Hour(temp_start) < Dates.Hour(12)
HH2 = Dates.Hour(12)
elseif Dates.Hour(12) <= Dates.Hour(temp_start) < Dates.Hour(16)
HH2 = Dates.Hour(16)
elseif Dates.Hour(16) <= Dates.Hour(temp_start) < Dates.Hour(20)
HH2 = Dates.Hour(20)
elseif Dates.Hour(20) <= Dates.Hour(temp_start) < Dates.Hour(24)
HH2 = Dates.Hour(0)
DD2 = DD2 + Dates.Day(1)
end
next_start = DateTime(Dates.Year(temp_start),Dates.Month(temp_start),DD2,HH2)
# Generate File Name
for j=1:1:length(header)
if occursin(r"^Instrument:",header[j])
fname = joinpath(Dest,Dates.format(temp_start,"yyyy-mm-ddTHHMMSS") * "_" * header[j][13:end-1] * ".data")
end
end
if verbose
println("\t\t- " * fname)
end
fid2 = open(fname,"w+")
# Find and replace the Timestamp header line
for j=1:1:length(header);
if occursin(r"^Timestamp",header[j])
header[j] = "Timestamp: " * Dates.format(temp_start,"yyyy-mm-dd HH:MM:SS:sss") * "\n"
end
end
# Iteratively write the header
for j=1:1:length(header)
write(fid2,header[j])
end
write(fid2,l) # Write the line used to create the file name
else
# An output stream is available
l = readline(fid,keep=true) # Load in another line
# Write or Close
ft = findall(x -> x == '\t',l)
temp_end = DateTime(l[ft[6]+1:ft[8]-1],"yyyy-mm-dd\tHH:MM:SS:sss")
if temp_end >= next_start
# The current line is newer than the start of the next file, close the current file
close(fid2)
# Zipped File Name (minus extension)
fzip = splitext(fname)[1]
# Zip File
if verbose
println("\t\t\tCompressing...")
end
fzip = fzip * ".ghg"
ziptextfiles(fzip,fname) # Zip file
rm(fname)
else
# Still within range, write the current line
write(fid2,l)
end
end
end
close(fid2)
close(fid)
# Zipped File Name (minus extension)
fzip = splitext(fname)[1]
# Zip the final file
if verbose
println("\t\t\tCompressing...")
end
fzip = fzip * ".ghg"
fzip2 = fzip * ".ghg"
ziptextfiles(fzip,fname) # Zip file
rm(fname)
end
if verbose
println("Complete")
end
end
| [
2,
3476,
273,
62,
35312,
13,
20362,
201,
198,
2,
201,
198,
2,
220,
220,
27758,
7455,
12,
22,
2167,
15326,
51,
3696,
656,
24739,
38,
22415,
201,
198,
2,
201,
198,
2,
11753,
371,
9116,
36761,
201,
198,
2,
536,
9116,
38572,
37931,
315,
201,
198,
2,
37931,
315,
277,
25151,
317,
2164,
668,
2475,
292,
354,
27839,
201,
198,
2,
20000,
4992,
4912,
399,
2043,
49,
2640,
11909,
9338,
201,
198,
2,
22300,
657,
13,
22,
201,
198,
2,
1160,
13,
2713,
13,
4967,
201,
198,
2,
4586,
5312,
25,
1105,
13,
3023,
13,
23344,
201,
198,
201,
198,
1,
2,
3476,
273,
62,
35312,
7,
10459,
3712,
10100,
11,
16520,
1883,
3712,
10100,
26,
19011,
577,
3712,
33,
970,
28,
9562,
8,
201,
198,
201,
198,
41205,
281,
7455,
12,
22,
2167,
2420,
2393,
656,
1402,
3696,
287,
262,
3210,
24739,
38,
5794,
13,
1002,
1744,
11,
3696,
481,
923,
319,
262,
6678,
1440,
1711,
8849,
357,
2388,
11,
657,
7029,
11,
657,
7410,
11,
3503,
15729,
201,
198,
201,
198,
63,
677,
273,
62,
35312,
7,
10459,
11,
16520,
1883,
8,
63,
27758,
7455,
22,
2167,
3696,
287,
262,
2723,
13445,
59,
77,
201,
198,
9,
12429,
10459,
1174,
3712,
10100,
796,
27387,
286,
7455,
12,
22,
2167,
2420,
3696,
351,
3891,
2092,
284,
1584,
12,
3070,
12,
1558,
51,
940,
2231,
405,
13,
14116,
201,
198,
9,
12429,
16520,
1883,
1174,
3712,
10100,
796,
45657,
8619,
59,
77,
59,
77,
201,
198,
201,
198,
6329,
59,
77,
201,
198,
201,
198,
4242,
7383,
10879,
7479,
77,
201,
198,
9,
15942,
577,
3712,
33,
970,
796,
16531,
1321,
355,
262,
2163,
4539,
11,
26751,
318,
4277,
201,
198,
59,
77,
59,
77,
1,
201,
198,
8818,
3476,
273,
62,
35312,
7,
6187,
3712,
10100,
11,
24159,
3712,
10100,
26,
19011,
577,
3712,
33,
970,
28,
7942,
8,
201,
198,
220,
220,
220,
1303,
14468,
2235,
201,
198,
220,
220,
220,
22492,
220,
45205,
220,
22492,
201,
198,
220,
220,
220,
1303,
14468,
2235,
201,
198,
220,
220,
220,
1303,
1583,
796,
8090,
27387,
201,
198,
220,
220,
220,
1303,
8145,
796,
45657,
27387,
201,
198,
197,
201,
198,
220,
220,
220,
1303,
14468,
21017,
201,
198,
220,
220,
220,
22492,
220,
2547,
325,
23412,
82,
220,
22492,
201,
198,
220,
220,
220,
1303,
14468,
21017,
201,
198,
220,
220,
220,
1303,
6822,
27387,
3254,
17995,
201,
198,
220,
220,
220,
611,
5299,
9409,
343,
7,
6187,
8,
930,
5299,
9409,
343,
7,
24159,
8,
201,
198,
220,
220,
220,
220,
220,
220,
220,
4049,
7203,
7416,
393,
10965,
8619,
857,
407,
2152,
4943,
201,
198,
220,
220,
220,
886,
201,
198,
197,
201,
198,
220,
220,
220,
357,
16624,
11,
11379,
364,
8,
796,
288,
1901,
396,
7,
6187,
11,
260,
25636,
28,
81,
1,
59,
67,
90,
19,
92,
12,
59,
67,
90,
17,
92,
12,
59,
67,
90,
17,
92,
51,
59,
67,
90,
21,
92,
17405,
14116,
3,
1600,
8344,
333,
28,
16,
8,
1303,
7343,
15326,
51,
3696,
201,
198,
197,
201,
198,
220,
220,
220,
1303,
14468,
4242,
201,
198,
220,
220,
220,
22492,
220,
10854,
13283,
220,
22492,
201,
198,
220,
220,
220,
1303,
14468,
4242,
201,
198,
220,
220,
220,
611,
318,
28920,
7,
16624,
8,
201,
198,
197,
220,
220,
220,
44872,
7203,
2949,
3395,
7890,
3696,
284,
6626,
4943,
201,
198,
220,
220,
220,
2073,
201,
198,
220,
220,
220,
220,
197,
361,
15942,
577,
201,
198,
197,
220,
220,
220,
220,
197,
35235,
7203,
18604,
13341,
2535,
3395,
7890,
13283,
24844,
4943,
201,
198,
197,
220,
220,
220,
886,
201,
198,
220,
220,
220,
886,
201,
198,
220,
220,
220,
640,
796,
7536,
7575,
21737,
201,
198,
220,
220,
220,
923,
2435,
796,
7536,
7575,
21737,
201,
198,
220,
220,
220,
886,
2435,
796,
7536,
7575,
21737,
201,
198,
220,
220,
220,
277,
3672,
796,
10903,
21737,
201,
198,
220,
220,
220,
13639,
796,
15690,
90,
10100,
92,
7,
917,
891,
11,
15,
8,
1303,
554,
13562,
13639,
7177,
201,
198,
220,
220,
220,
1908,
20538,
796,
1160,
26,
1303,
26716,
1988,
329,
13639,
981,
9052,
201,
198,
220,
220,
220,
329,
1312,
28,
16,
25,
16,
25,
13664,
7,
16624,
8,
201,
198,
220,
220,
220,
220,
197,
361,
15942,
577,
201,
198,
197,
220,
220,
220,
220,
197,
35235,
7203,
220,
220,
366,
1635,
3696,
58,
72,
12962,
201,
198,
197,
220,
220,
220,
886,
201,
198,
197,
220,
220,
220,
264,
796,
1185,
7,
16624,
58,
72,
12962,
1303,
3497,
2393,
7508,
201,
198,
197,
197,
201,
198,
197,
220,
220,
220,
49909,
796,
1280,
7,
16624,
58,
72,
17241,
81,
4943,
201,
198,
197,
197,
201,
198,
197,
220,
220,
220,
1303,
8778,
48900,
201,
198,
197,
220,
220,
220,
474,
796,
352,
26,
201,
198,
197,
220,
220,
220,
300,
796,
366,
33,
959,
6607,
1,
1303,
36557,
597,
4731,
379,
1551,
642,
3435,
890,
201,
198,
197,
220,
220,
220,
981,
300,
58,
16,
25,
20,
60,
14512,
366,
26947,
39,
1,
11405,
474,
1279,
1908,
20538,
201,
198,
197,
220,
220,
220,
220,
197,
75,
796,
1100,
1370,
7,
69,
312,
11,
14894,
28,
7942,
8,
201,
198,
197,
220,
220,
220,
220,
197,
25677,
796,
685,
25677,
26,
75,
60,
201,
198,
197,
220,
220,
220,
220,
197,
73,
15853,
352,
201,
198,
197,
220,
220,
220,
886,
201,
198,
197,
220,
220,
220,
1366,
62,
1930,
796,
2292,
7,
69,
312,
8,
1303,
23158,
286,
810,
262,
1366,
4940,
201,
198,
197,
197,
201,
198,
197,
220,
220,
220,
1303,
3855,
7253,
3862,
201,
198,
197,
220,
220,
220,
300,
796,
1100,
1370,
7,
69,
312,
11,
14894,
28,
7942,
8,
201,
198,
197,
197,
701,
796,
1064,
439,
7,
87,
4613,
2124,
6624,
705,
59,
83,
3256,
75,
8,
201,
198,
197,
220,
220,
220,
923,
2435,
796,
7536,
7575,
7,
75,
58,
701,
58,
21,
48688,
16,
25,
701,
58,
23,
45297,
16,
17241,
22556,
22556,
12,
3020,
12,
1860,
59,
83,
16768,
25,
12038,
25,
5432,
25,
824,
82,
4943,
201,
198,
197,
197,
201,
198,
197,
220,
220,
220,
1303,
3497,
5268,
3862,
201,
198,
197,
220,
220,
220,
886,
1370,
796,
2081,
201,
198,
197,
220,
220,
220,
938,
1930,
796,
604,
1303,
26813,
422,
262,
886,
286,
262,
2393,
810,
262,
938,
1627,
6140,
201,
198,
197,
220,
220,
220,
981,
886,
1370,
6624,
2081,
201,
198,
197,
197,
220,
220,
220,
5380,
7,
69,
312,
11,
82,
13,
7857,
12,
12957,
1930,
8,
201,
198,
197,
197,
220,
220,
220,
300,
796,
1100,
1370,
7,
69,
312,
11,
14894,
28,
7942,
8,
201,
198,
197,
197,
220,
220,
220,
611,
304,
1659,
7,
69,
312,
8,
6624,
3991,
201,
198,
197,
197,
197,
220,
220,
220,
886,
1370,
796,
3991,
201,
198,
197,
197,
220,
220,
220,
2073,
201,
198,
197,
197,
197,
220,
220,
220,
938,
1930,
15853,
362,
201,
198,
197,
197,
220,
220,
220,
886,
201,
198,
197,
220,
220,
220,
886,
201,
198,
197,
220,
220,
220,
300,
796,
1100,
1370,
7,
69,
312,
11,
14894,
28,
7942,
8,
201,
198,
197,
197,
701,
796,
1064,
439,
7,
87,
4613,
2124,
6624,
705,
59,
83,
3256,
75,
8,
201,
198,
197,
220,
220,
220,
886,
2435,
796,
7536,
7575,
7,
75,
58,
701,
58,
21,
48688,
16,
25,
701,
58,
23,
45297,
16,
17241,
22556,
22556,
12,
3020,
12,
1860,
59,
83,
16768,
25,
12038,
25,
5432,
25,
824,
82,
4943,
201,
198,
197,
197,
201,
198,
197,
220,
220,
220,
1303,
27758,
262,
9220,
201,
198,
197,
220,
220,
220,
5380,
7,
69,
312,
11,
7890,
62,
1930,
8,
1303,
10028,
262,
23493,
284,
262,
923,
286,
262,
1366,
201,
198,
197,
220,
220,
220,
49909,
17,
796,
1280,
7,
16624,
58,
72,
36563,
19836,
7,
69,
312,
17,
8,
201,
198,
197,
220,
220,
220,
1306,
62,
9688,
796,
17635,
201,
198,
197,
220,
220,
220,
717,
62,
7753,
796,
2081,
201,
198,
197,
220,
220,
220,
981,
304,
1659,
7,
69,
312,
8,
6624,
3991,
201,
198,
197,
197,
220,
220,
220,
611,
318,
9654,
7,
69,
312,
17,
8,
6624,
3991,
201,
198,
197,
197,
197,
220,
220,
220,
1303,
1400,
5072,
4269,
1695,
201,
198,
197,
197,
197,
220,
220,
220,
1303,
4946,
649,
5072,
2393,
422,
2180,
1627,
201,
198,
197,
197,
197,
197,
201,
198,
197,
197,
197,
220,
220,
220,
611,
717,
62,
7753,
6624,
2081,
201,
198,
197,
197,
197,
197,
220,
220,
220,
1303,
1318,
318,
407,
4271,
9639,
1627,
11,
3440,
530,
783,
201,
198,
197,
197,
197,
197,
220,
220,
220,
300,
796,
1100,
1370,
7,
69,
312,
11,
14894,
28,
7942,
8,
201,
198,
197,
197,
197,
197,
220,
220,
220,
717,
62,
7753,
796,
3991,
201,
198,
197,
197,
197,
220,
220,
220,
886,
201,
198,
197,
197,
197,
197,
197,
201,
198,
197,
197,
197,
220,
220,
220,
1303,
27131,
378,
938,
1988,
286,
2393,
201,
198,
197,
197,
197,
197,
701,
796,
1064,
439,
7,
87,
4613,
2124,
6624,
705,
59,
83,
3256,
75,
8,
201,
198,
197,
197,
197,
220,
220,
220,
20218,
62,
9688,
796,
7536,
7575,
7,
75,
58,
701,
58,
21,
48688,
16,
25,
701,
58,
23,
45297,
16,
17241,
22556,
22556,
12,
3020,
12,
1860,
59,
83,
16768,
25,
12038,
25,
5432,
25,
824,
82,
4943,
201,
198,
197,
197,
197,
220,
220,
220,
47138,
17,
796,
44712,
13,
43223,
21737,
201,
198,
197,
197,
197,
220,
220,
220,
20084,
17,
796,
44712,
13,
12393,
7,
29510,
62,
9688,
8,
201,
198,
197,
197,
197,
220,
220,
220,
611,
44712,
13,
43223,
7,
15,
8,
19841,
44712,
13,
43223,
7,
29510,
62,
9688,
8,
1279,
44712,
13,
43223,
7,
19,
8,
201,
198,
197,
197,
197,
197,
220,
220,
220,
47138,
17,
796,
44712,
13,
43223,
7,
19,
8,
201,
198,
197,
197,
197,
220,
220,
220,
2073,
361,
44712,
13,
43223,
7,
19,
8,
19841,
44712,
13,
43223,
7,
29510,
62,
9688,
8,
1279,
44712,
13,
43223,
7,
23,
8,
201,
198,
197,
197,
197,
197,
220,
220,
220,
47138,
17,
796,
44712,
13,
43223,
7,
23,
8,
201,
198,
197,
197,
197,
220,
220,
220,
2073,
361,
44712,
13,
43223,
7,
23,
8,
19841,
44712,
13,
43223,
7,
29510,
62,
9688,
8,
1279,
44712,
13,
43223,
7,
1065,
8,
201,
198,
197,
197,
197,
197,
220,
220,
220,
47138,
17,
796,
44712,
13,
43223,
7,
1065,
8,
201,
198,
197,
197,
197,
220,
220,
220,
2073,
361,
44712,
13,
43223,
7,
1065,
8,
19841,
44712,
13,
43223,
7,
29510,
62,
9688,
8,
1279,
44712,
13,
43223,
7,
1433,
8,
201,
198,
197,
197,
197,
197,
220,
220,
220,
47138,
17,
796,
44712,
13,
43223,
7,
1433,
8,
201,
198,
197,
197,
197,
220,
220,
220,
2073,
361,
44712,
13,
43223,
7,
1433,
8,
19841,
44712,
13,
43223,
7,
29510,
62,
9688,
8,
1279,
44712,
13,
43223,
7,
1238,
8,
201,
198,
197,
197,
197,
197,
220,
220,
220,
47138,
17,
796,
44712,
13,
43223,
7,
1238,
8,
201,
198,
197,
197,
197,
220,
220,
220,
2073,
361,
44712,
13,
43223,
7,
1238,
8,
19841,
44712,
13,
43223,
7,
29510,
62,
9688,
8,
1279,
44712,
13,
43223,
7,
1731,
8,
201,
198,
197,
197,
197,
197,
220,
220,
220,
47138,
17,
796,
44712,
13,
43223,
7,
15,
8,
201,
198,
197,
197,
197,
197,
220,
220,
220,
20084,
17,
796,
20084,
17,
1343,
44712,
13,
12393,
7,
16,
8,
201,
198,
197,
197,
197,
220,
220,
220,
886,
201,
198,
197,
197,
197,
220,
220,
220,
1306,
62,
9688,
796,
7536,
7575,
7,
35,
689,
13,
17688,
7,
29510,
62,
9688,
828,
35,
689,
13,
31948,
7,
29510,
62,
9688,
828,
16458,
17,
11,
16768,
17,
8,
201,
198,
197,
197,
197,
197,
201,
198,
197,
197,
197,
220,
220,
220,
1303,
2980,
378,
9220,
6530,
201,
198,
197,
197,
197,
220,
220,
220,
329,
474,
28,
16,
25,
16,
25,
13664,
7,
25677,
8,
201,
198,
197,
197,
197,
220,
220,
220,
220,
197,
361,
8833,
259,
7,
81,
1,
61,
818,
43872,
25,
1600,
25677,
58,
73,
12962,
201,
198,
197,
197,
197,
220,
220,
220,
220,
197,
197,
69,
3672,
796,
4654,
6978,
7,
24159,
11,
35,
689,
13,
18982,
7,
29510,
62,
9688,
553,
22556,
22556,
12,
3020,
12,
1860,
4221,
39,
12038,
5432,
4943,
1635,
45434,
1,
1635,
13639,
58,
73,
7131,
1485,
25,
437,
12,
16,
60,
1635,
27071,
7890,
4943,
201,
198,
197,
197,
197,
220,
220,
220,
220,
197,
437,
201,
198,
197,
197,
197,
197,
437,
201,
198,
197,
197,
197,
197,
361,
15942,
577,
201,
198,
197,
197,
197,
220,
220,
220,
220,
197,
35235,
7203,
59,
83,
59,
83,
12,
366,
1635,
277,
3672,
8,
201,
198,
197,
197,
197,
220,
220,
220,
886,
201,
198,
197,
197,
197,
220,
220,
220,
49909,
17,
796,
1280,
7,
69,
3672,
553,
86,
10,
4943,
201,
198,
197,
197,
197,
197,
201,
198,
197,
197,
197,
220,
220,
220,
1303,
9938,
290,
6330,
262,
5045,
27823,
13639,
1627,
201,
198,
197,
197,
197,
220,
220,
220,
329,
474,
28,
16,
25,
16,
25,
13664,
7,
25677,
1776,
201,
198,
197,
197,
197,
220,
220,
220,
220,
197,
361,
8833,
259,
7,
81,
1,
61,
14967,
27823,
1600,
25677,
58,
73,
12962,
201,
198,
197,
197,
197,
220,
220,
220,
220,
197,
197,
25677,
58,
73,
60,
796,
366,
14967,
27823,
25,
220,
366,
1635,
44712,
13,
18982,
7,
29510,
62,
9688,
553,
22556,
22556,
12,
3020,
12,
1860,
47138,
25,
12038,
25,
5432,
25,
824,
82,
4943,
1635,
37082,
77,
1,
201,
198,
197,
197,
197,
220,
220,
220,
220,
197,
437,
201,
198,
197,
197,
197,
220,
220,
220,
886,
201,
198,
197,
197,
197,
197,
201,
198,
197,
197,
197,
220,
220,
220,
1303,
40806,
9404,
3551,
262,
13639,
201,
198,
197,
197,
197,
220,
220,
220,
329,
474,
28,
16,
25,
16,
25,
13664,
7,
25677,
8,
201,
198,
197,
197,
197,
220,
220,
220,
220,
197,
13564,
7,
69,
312,
17,
11,
25677,
58,
73,
12962,
201,
198,
197,
197,
197,
220,
220,
220,
886,
201,
198,
197,
197,
197,
197,
201,
198,
197,
197,
197,
220,
220,
220,
3551,
7,
69,
312,
17,
11,
75,
8,
1303,
19430,
262,
1627,
973,
284,
2251,
262,
2393,
1438,
201,
198,
197,
197,
220,
220,
220,
2073,
201,
198,
197,
197,
197,
220,
220,
220,
1303,
1052,
5072,
4269,
318,
1695,
201,
198,
197,
197,
197,
220,
220,
220,
300,
796,
1100,
1370,
7,
69,
312,
11,
14894,
28,
7942,
8,
1303,
8778,
287,
1194,
1627,
201,
198,
197,
197,
197,
197,
201,
198,
197,
197,
197,
220,
220,
220,
1303,
19430,
393,
13872,
201,
198,
197,
197,
197,
197,
701,
796,
1064,
439,
7,
87,
4613,
2124,
6624,
705,
59,
83,
3256,
75,
8,
201,
198,
197,
197,
197,
220,
220,
220,
20218,
62,
437,
796,
7536,
7575,
7,
75,
58,
701,
58,
21,
48688,
16,
25,
701,
58,
23,
45297,
16,
17241,
22556,
22556,
12,
3020,
12,
1860,
59,
83,
16768,
25,
12038,
25,
5432,
25,
824,
82,
4943,
201,
198,
197,
197,
197,
220,
220,
220,
611,
20218,
62,
437,
18189,
1306,
62,
9688,
201,
198,
197,
197,
197,
197,
220,
220,
220,
1303,
383,
1459,
1627,
318,
15064,
621,
262,
923,
286,
262,
1306,
2393,
11,
1969,
262,
1459,
2393,
201,
198,
197,
197,
197,
197,
220,
220,
220,
1969,
7,
69,
312,
17,
8,
201,
198,
197,
197,
197,
197,
197,
201,
198,
197,
197,
197,
197,
220,
220,
220,
1303,
1168,
3949,
9220,
6530,
357,
40191,
7552,
8,
201,
198,
197,
197,
197,
197,
220,
220,
220,
277,
13344,
796,
4328,
578,
742,
7,
69,
3672,
38381,
16,
60,
201,
198,
197,
197,
197,
197,
197,
201,
198,
197,
197,
197,
197,
220,
220,
220,
1303,
38636,
9220,
201,
198,
197,
197,
197,
197,
220,
220,
220,
611,
15942,
577,
201,
198,
197,
197,
197,
197,
220,
220,
220,
220,
197,
35235,
7203,
59,
83,
59,
83,
59,
83,
7293,
11697,
9313,
8,
201,
198,
197,
197,
197,
197,
220,
220,
220,
886,
201,
198,
197,
197,
197,
197,
220,
220,
220,
277,
13344,
796,
277,
13344,
1635,
27071,
456,
70,
1,
201,
198,
197,
197,
197,
197,
197,
89,
10257,
2302,
16624,
7,
69,
13344,
11,
69,
3672,
8,
1303,
38636,
2393,
201,
198,
197,
197,
197,
197,
220,
220,
220,
42721,
7,
69,
3672,
8,
201,
198,
197,
197,
197,
220,
220,
220,
2073,
201,
198,
197,
197,
197,
197,
220,
220,
220,
1303,
7831,
1626,
2837,
11,
3551,
262,
1459,
1627,
201,
198,
197,
197,
197,
197,
220,
220,
220,
3551,
7,
69,
312,
17,
11,
75,
8,
201,
198,
197,
197,
197,
220,
220,
220,
886,
201,
198,
197,
197,
220,
220,
220,
886,
201,
198,
197,
220,
220,
220,
886,
201,
198,
197,
197,
201,
198,
197,
220,
220,
220,
1969,
7,
69,
312,
17,
8,
201,
198,
197,
220,
220,
220,
1969,
7,
69,
312,
8,
201,
198,
197,
197,
201,
198,
197,
197,
2,
1168,
3949,
9220,
6530,
357,
40191,
7552,
8,
201,
198,
197,
197,
69,
13344,
796,
4328,
578,
742,
7,
69,
3672,
38381,
16,
60,
201,
198,
197,
197,
201,
198,
197,
197,
2,
38636,
262,
2457,
2393,
201,
198,
197,
197,
361,
15942,
577,
201,
198,
197,
197,
197,
35235,
7203,
59,
83,
59,
83,
59,
83,
7293,
11697,
9313,
8,
201,
198,
197,
197,
437,
201,
198,
197,
197,
69,
13344,
796,
277,
13344,
1635,
27071,
456,
70,
1,
201,
198,
197,
220,
220,
220,
277,
13344,
17,
796,
277,
13344,
1635,
27071,
456,
70,
1,
201,
198,
197,
197,
89,
10257,
2302,
16624,
7,
69,
13344,
11,
69,
3672,
8,
1303,
38636,
2393,
201,
198,
197,
220,
220,
220,
42721,
7,
69,
3672,
8,
201,
198,
220,
220,
220,
886,
201,
198,
197,
201,
198,
220,
220,
220,
611,
15942,
577,
201,
198,
220,
220,
220,
220,
197,
35235,
7203,
20988,
4943,
201,
198,
220,
220,
220,
886,
201,
198,
437,
201,
198
] | 2.100363 | 3,029 |
function CircularFieldOfView(arg0::Vector3D, arg1::jdouble, arg2::jdouble)
return CircularFieldOfView((Vector3D, jdouble, jdouble), arg0, arg1, arg2)
end
function get_center(obj::SmoothFieldOfView)
return jcall(obj, "getCenter", Vector3D, ())
end
function get_footprint(obj::SmoothFieldOfView, arg0::Transform, arg1::OneAxisEllipsoid, arg2::jdouble)
return jcall(obj, "getFootprint", List, (Transform, OneAxisEllipsoid, jdouble), arg0, arg1, arg2)
end
function get_half_aperture(obj::CircularFieldOfView)
return jcall(obj, "getHalfAperture", jdouble, ())
end
function get_x(obj::SmoothFieldOfView)
return jcall(obj, "getX", Vector3D, ())
end
function get_y(obj::SmoothFieldOfView)
return jcall(obj, "getY", Vector3D, ())
end
function get_z(obj::SmoothFieldOfView)
return jcall(obj, "getZ", Vector3D, ())
end
function offset_from_boundary(obj::CircularFieldOfView, arg0::Vector3D, arg1::jdouble, arg2::VisibilityTrigger)
return jcall(obj, "offsetFromBoundary", jdouble, (Vector3D, jdouble, VisibilityTrigger), arg0, arg1, arg2)
end
function project_to_boundary(obj::CircularFieldOfView, arg0::Vector3D)
return jcall(obj, "projectToBoundary", Vector3D, (Vector3D,), arg0)
end
| [
8818,
7672,
934,
15878,
5189,
7680,
7,
853,
15,
3712,
38469,
18,
35,
11,
1822,
16,
3712,
73,
23352,
11,
1822,
17,
3712,
73,
23352,
8,
198,
220,
220,
220,
1441,
7672,
934,
15878,
5189,
7680,
19510,
38469,
18,
35,
11,
474,
23352,
11,
474,
23352,
828,
1822,
15,
11,
1822,
16,
11,
1822,
17,
8,
198,
437,
198,
198,
8818,
651,
62,
16159,
7,
26801,
3712,
7556,
5226,
15878,
5189,
7680,
8,
198,
220,
220,
220,
1441,
474,
13345,
7,
26801,
11,
366,
1136,
23656,
1600,
20650,
18,
35,
11,
32865,
198,
437,
198,
198,
8818,
651,
62,
5898,
4798,
7,
26801,
3712,
7556,
5226,
15878,
5189,
7680,
11,
1822,
15,
3712,
41762,
11,
1822,
16,
3712,
3198,
31554,
271,
30639,
541,
568,
312,
11,
1822,
17,
3712,
73,
23352,
8,
198,
220,
220,
220,
1441,
474,
13345,
7,
26801,
11,
366,
1136,
17574,
4798,
1600,
7343,
11,
357,
41762,
11,
1881,
31554,
271,
30639,
541,
568,
312,
11,
474,
23352,
828,
1822,
15,
11,
1822,
16,
11,
1822,
17,
8,
198,
437,
198,
198,
8818,
651,
62,
13959,
62,
499,
861,
495,
7,
26801,
3712,
31560,
934,
15878,
5189,
7680,
8,
198,
220,
220,
220,
1441,
474,
13345,
7,
26801,
11,
366,
1136,
31305,
32,
27286,
1600,
474,
23352,
11,
32865,
198,
437,
198,
198,
8818,
651,
62,
87,
7,
26801,
3712,
7556,
5226,
15878,
5189,
7680,
8,
198,
220,
220,
220,
1441,
474,
13345,
7,
26801,
11,
366,
1136,
55,
1600,
20650,
18,
35,
11,
32865,
198,
437,
198,
198,
8818,
651,
62,
88,
7,
26801,
3712,
7556,
5226,
15878,
5189,
7680,
8,
198,
220,
220,
220,
1441,
474,
13345,
7,
26801,
11,
366,
1136,
56,
1600,
20650,
18,
35,
11,
32865,
198,
437,
198,
198,
8818,
651,
62,
89,
7,
26801,
3712,
7556,
5226,
15878,
5189,
7680,
8,
198,
220,
220,
220,
1441,
474,
13345,
7,
26801,
11,
366,
1136,
57,
1600,
20650,
18,
35,
11,
32865,
198,
437,
198,
198,
8818,
11677,
62,
6738,
62,
7784,
560,
7,
26801,
3712,
31560,
934,
15878,
5189,
7680,
11,
1822,
15,
3712,
38469,
18,
35,
11,
1822,
16,
3712,
73,
23352,
11,
1822,
17,
3712,
15854,
2247,
48344,
8,
198,
220,
220,
220,
1441,
474,
13345,
7,
26801,
11,
366,
28968,
4863,
49646,
560,
1600,
474,
23352,
11,
357,
38469,
18,
35,
11,
474,
23352,
11,
6911,
2247,
48344,
828,
1822,
15,
11,
1822,
16,
11,
1822,
17,
8,
198,
437,
198,
198,
8818,
1628,
62,
1462,
62,
7784,
560,
7,
26801,
3712,
31560,
934,
15878,
5189,
7680,
11,
1822,
15,
3712,
38469,
18,
35,
8,
198,
220,
220,
220,
1441,
474,
13345,
7,
26801,
11,
366,
16302,
2514,
49646,
560,
1600,
20650,
18,
35,
11,
357,
38469,
18,
35,
11,
828,
1822,
15,
8,
198,
437,
628
] | 2.65 | 460 |
using Cairo
using LSystems
c = CairoRGBSurface(485, 720);
cr = CairoContext(c);
save(cr);
set_source_rgb(cr, 1.0, 1.0, 1.0);
rectangle(cr, 0.0, 0.0, 485.0, 720.0);
fill(cr);
restore(cr);
save(cr);
x, y, a, d = 310., 560., 90, 15
set_line_width(cr, 1);
move_to(cr, x, y);
dragon_start = "A"
dragon_trans = Dict([
('A', "A+BF"),
('B', "FA-B")
])
function determine_new_position(x, y, a, d)
x -= sind(a) * d
y -= cosd(a) * d
return x, y
end
level = 10
drawing_instructions = @task dol_system(dragon_start, dragon_trans, level)
for v in drawing_instructions
if v == 'F'
x, y = determine_new_position(x, y, a, d)
line_to(cr, x, y)
elseif v == '+'
a -= 90
elseif v == '-'
a += 90
elseif v == '['
push!(s, [x, y, a])
elseif v == ']'
x, y, a = pop!(s)
move_to(cr, x, y)
end
end
stroke(cr);
write_to_png(c, "dragon_$level.png");
| [
3500,
23732,
198,
3500,
406,
11964,
82,
198,
198,
66,
796,
23732,
48192,
4462,
333,
2550,
7,
32642,
11,
26250,
1776,
198,
6098,
796,
23732,
21947,
7,
66,
1776,
198,
198,
21928,
7,
6098,
1776,
198,
198,
2617,
62,
10459,
62,
81,
22296,
7,
6098,
11,
352,
13,
15,
11,
352,
13,
15,
11,
352,
13,
15,
1776,
198,
2554,
9248,
7,
6098,
11,
657,
13,
15,
11,
657,
13,
15,
11,
4764,
20,
13,
15,
11,
26250,
13,
15,
1776,
198,
20797,
7,
6098,
1776,
198,
198,
2118,
382,
7,
6098,
1776,
198,
21928,
7,
6098,
1776,
198,
198,
87,
11,
331,
11,
257,
11,
288,
796,
28947,
1539,
38089,
1539,
4101,
11,
1315,
198,
2617,
62,
1370,
62,
10394,
7,
6098,
11,
352,
1776,
198,
21084,
62,
1462,
7,
6098,
11,
2124,
11,
331,
1776,
198,
198,
14844,
62,
9688,
796,
366,
32,
1,
198,
14844,
62,
7645,
796,
360,
713,
26933,
198,
10786,
32,
3256,
366,
32,
10,
29499,
12340,
198,
10786,
33,
3256,
366,
7708,
12,
33,
4943,
198,
12962,
198,
198,
8818,
5004,
62,
3605,
62,
9150,
7,
87,
11,
331,
11,
257,
11,
288,
8,
198,
220,
220,
220,
2124,
48185,
264,
521,
7,
64,
8,
1635,
288,
198,
220,
220,
220,
331,
48185,
8615,
67,
7,
64,
8,
1635,
288,
198,
220,
220,
220,
1441,
2124,
11,
331,
198,
437,
198,
198,
5715,
796,
838,
198,
19334,
278,
62,
259,
7249,
507,
796,
2488,
35943,
288,
349,
62,
10057,
7,
14844,
62,
9688,
11,
10441,
62,
7645,
11,
1241,
8,
198,
198,
1640,
410,
287,
8263,
62,
259,
7249,
507,
198,
220,
220,
220,
611,
410,
6624,
705,
37,
6,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
11,
331,
796,
5004,
62,
3605,
62,
9150,
7,
87,
11,
331,
11,
257,
11,
288,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1627,
62,
1462,
7,
6098,
11,
2124,
11,
331,
8,
198,
220,
220,
220,
2073,
361,
410,
6624,
705,
10,
6,
198,
220,
220,
220,
220,
220,
220,
220,
257,
48185,
4101,
198,
220,
220,
220,
2073,
361,
410,
6624,
705,
19355,
198,
220,
220,
220,
220,
220,
220,
220,
257,
15853,
4101,
198,
220,
220,
220,
2073,
361,
410,
6624,
705,
17816,
198,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
82,
11,
685,
87,
11,
331,
11,
257,
12962,
198,
220,
220,
220,
2073,
361,
410,
6624,
705,
49946,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
11,
331,
11,
257,
796,
1461,
0,
7,
82,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1445,
62,
1462,
7,
6098,
11,
2124,
11,
331,
8,
198,
220,
220,
220,
886,
198,
437,
198,
198,
30757,
7,
6098,
1776,
198,
13564,
62,
1462,
62,
11134,
7,
66,
11,
366,
14844,
62,
3,
5715,
13,
11134,
15341,
198
] | 1.95339 | 472 |
###########
## asArr ##
###########
## asArr: try conversion to Array, implicitly dealing with NAs
##
## using DataFrames, columns may be of different type and hence are
## possibly not compatible with each other as columns of an array
function asArr{T}(da::DataArray{T, 1}, typ::Type=Any,
replaceNA=NA)
## convert DataArray to typ and replace NAs with replaceNA
vals = convert(Array{typ, 1}, da.data)
vals[da.na] = replaceNA
return vals
end
function asArr(df::DataFrame, typ::Type=Any,
replaceNA=NA)
nObs, nAss = size(df)
vals = Array(typ, nObs, nAss)
## copying into this array implicitly forces conversion to this
## type afterwards
for ii=1:nAss
if isa(df.columns[ii], DataArray) # deal with NAs
if eltype(df.columns[ii]) == NAtype # column of NAs only
vals[:, ii] = replaceNA
else
vals[:, ii] = df.columns[ii].data
vals[df.columns[ii].na, ii] = replaceNA
end
else # no NAs in simple Array
vals[:, ii] = df.columns[ii]
end
end
return vals
end
function asArr(dfr::DataFrameRow, typ::Type=Any,
replaceNA=NA)
nObs = length(dfr)
res = Array(typ, 1, nObs)
for ii=1:nObs
if isna(dfr[ii])
res[1, ii] = replaceNA
else
res[1, ii] = dfr[ii]
end
end
return res
end
function asArr(tn::AbstractTimedata, typ::Type=Any,
replaceNA=NA)
return asArr(tn.vals, typ, replaceNA)
end
## problem: columns with NAs only
## problem: NaN in boolean context will be true
## problem: DataArray{Any, 1} also exist: da.data then contains NAs
#############
## Timenum ##
#############
import Base.convert
## to Timedata: conversion upwards - always works
function convert(::Type{Timedata}, tn::Timenum)
return Timedata(tn.vals, tn.idx)
end
## to Timematr: conversion downwards - fails for NAs
function convert(::Type{Timematr}, tn::Timenum, rmNA = false)
if rmNA
tm = narm(tn)
tm = Timematr(tm.vals, tm.idx)
else
tm = Timematr(tn.vals, tn.idx)
end
return tm
end
##############
## Timematr ##
##############
## to Timedata: conversion upwards - always works
function convert(::Type{Timedata}, tm::Timematr)
Timedata(tm.vals, tm.idx)
end
## to Timenum: conversion upwards - always works
function convert(::Type{Timenum}, tm::Timematr)
Timenum(tm.vals, tm.idx)
end
##############
## Timedata ##
##############
## to Timenum: conversion downwards - fails for non-numeric values
function convert(::Type{Timenum}, td::Timedata)
Timenum(td.vals, td.idx)
end
## to Timematr: conversion downwards - fails for NAs
function convert(::Type{Timematr}, td::Timedata)
Timematr(td.vals, td.idx)
end
################
## DataFrames ##
################
## convert DataFrame with dates column (as String) to TimeData object
function convert(::Type{AbstractTimedata}, df::DataFrame)
## test if some column already is of type Date
if any(eltypes(df) .== Date)
## take first occuring column
dateCol = find(eltypes(df) .== Date)[1]
idx = convert(Array, df[dateCol])
delete!(df, [dateCol])
else ## find column that contain dates as String
# find columns that have been parsed as Strings by readtable
col_to_test = Array(Symbol, 0)
nCols = size(df, 2)
for ii=1:nCols
isa(df[1, ii], String)?
push!(col_to_test, names(df)[ii]):
nothing
end
# test each column's data to see if Datetime will parse it
col_that_pass = Array(Symbol, 0)
for colname in col_to_test
d = match(r"[-|\s|\/|.]", df[1, colname])
d !== nothing? (bar = split(df[1, colname], d.match)): (bar = [])
if length(bar) == 3
push!(col_that_pass, colname)
end
end
# parse first column that passes the Datetime regex test
idx = Date[Date(d) for d in df[col_that_pass[1]]] # without
# Date it would fail chkIdx
# in constructor
delete!(df, [col_that_pass[1]])
end
## try whether DataFrame fits subtypes
try
td = Timematr(df, idx)
return td
catch
try
td = Timenum(df, idx)
return td
catch
td = Timedata(df, idx)
return td
end
end
return td
end
################
## TimeArrays ##
################
function convert(::Type{AbstractTimedata}, ta::TimeArray)
namesAsSymbols = [DataFrames.identifier(nam) for nam in ta.colnames]
df = composeDataFrame(ta.values, namesAsSymbols)
idx = ta.timestamp
try
td = Timematr(df, idx)
return td
catch
try
td = Timenum(df, idx)
return td
catch
td = Timedata(df, idx)
return td
end
end
td
end
function convert(::Type{TimeArray}, tn::AbstractTimenum)
dats = idx(tn)
nams = UTF8String[string(names(tn)[ii]) for ii = 1:size(tn, 2)]
return TimeArray(dats, asArr(tn, Float64, NaN), nams)
end
| [
7804,
21017,
198,
2235,
355,
3163,
81,
22492,
198,
7804,
21017,
198,
198,
2235,
355,
3163,
81,
25,
1949,
11315,
284,
15690,
11,
31821,
7219,
351,
399,
1722,
198,
2235,
220,
198,
2235,
1262,
6060,
35439,
11,
15180,
743,
307,
286,
1180,
2099,
290,
12891,
389,
198,
2235,
5457,
407,
11670,
351,
1123,
584,
355,
15180,
286,
281,
7177,
198,
198,
8818,
355,
3163,
81,
90,
51,
92,
7,
6814,
3712,
6601,
19182,
90,
51,
11,
352,
5512,
2170,
3712,
6030,
28,
7149,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6330,
4535,
28,
4535,
8,
198,
220,
220,
220,
22492,
10385,
6060,
19182,
284,
2170,
290,
6330,
399,
1722,
351,
6330,
4535,
198,
220,
220,
220,
410,
874,
796,
10385,
7,
19182,
90,
28004,
11,
352,
5512,
12379,
13,
7890,
8,
198,
220,
220,
220,
410,
874,
58,
6814,
13,
2616,
60,
796,
6330,
4535,
198,
220,
220,
220,
1441,
410,
874,
198,
437,
198,
198,
8818,
355,
3163,
81,
7,
7568,
3712,
6601,
19778,
11,
2170,
3712,
6030,
28,
7149,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6330,
4535,
28,
4535,
8,
198,
220,
220,
220,
299,
31310,
11,
299,
8021,
796,
2546,
7,
7568,
8,
198,
220,
220,
220,
410,
874,
796,
15690,
7,
28004,
11,
299,
31310,
11,
299,
8021,
8,
198,
220,
220,
220,
22492,
23345,
656,
428,
7177,
31821,
3386,
11315,
284,
428,
198,
220,
220,
220,
22492,
2099,
12979,
220,
198,
220,
220,
220,
329,
21065,
28,
16,
25,
77,
8021,
198,
220,
220,
220,
220,
220,
220,
220,
611,
318,
64,
7,
7568,
13,
28665,
82,
58,
4178,
4357,
6060,
19182,
8,
1303,
1730,
351,
399,
1722,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
1288,
4906,
7,
7568,
13,
28665,
82,
58,
4178,
12962,
6624,
11746,
4906,
1303,
5721,
286,
399,
1722,
691,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
410,
874,
58,
45299,
21065,
60,
796,
6330,
4535,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
410,
874,
58,
45299,
21065,
60,
796,
47764,
13,
28665,
82,
58,
4178,
4083,
7890,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
410,
874,
58,
7568,
13,
28665,
82,
58,
4178,
4083,
2616,
11,
21065,
60,
796,
6330,
4535,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
1303,
645,
399,
1722,
287,
2829,
15690,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
410,
874,
58,
45299,
21065,
60,
796,
47764,
13,
28665,
82,
58,
4178,
60,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
410,
874,
220,
220,
220,
220,
198,
437,
198,
198,
8818,
355,
3163,
81,
7,
7568,
81,
3712,
6601,
19778,
25166,
11,
2170,
3712,
6030,
28,
7149,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6330,
4535,
28,
4535,
8,
198,
220,
220,
220,
299,
31310,
796,
4129,
7,
7568,
81,
8,
198,
220,
220,
220,
581,
796,
15690,
7,
28004,
11,
352,
11,
299,
31310,
8,
198,
220,
220,
220,
329,
21065,
28,
16,
25,
77,
31310,
198,
220,
220,
220,
220,
220,
220,
220,
611,
2125,
64,
7,
7568,
81,
58,
4178,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
581,
58,
16,
11,
21065,
60,
796,
6330,
4535,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
581,
58,
16,
11,
21065,
60,
796,
288,
8310,
58,
4178,
60,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
581,
198,
437,
198,
198,
8818,
355,
3163,
81,
7,
34106,
3712,
23839,
14967,
276,
1045,
11,
2170,
3712,
6030,
28,
7149,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6330,
4535,
28,
4535,
8,
198,
220,
220,
220,
1441,
355,
3163,
81,
7,
34106,
13,
12786,
11,
2170,
11,
6330,
4535,
8,
198,
437,
198,
198,
2235,
1917,
25,
15180,
351,
399,
1722,
691,
198,
2235,
1917,
25,
11013,
45,
287,
25131,
4732,
481,
307,
2081,
198,
2235,
1917,
25,
6060,
19182,
90,
7149,
11,
352,
92,
635,
2152,
25,
12379,
13,
7890,
788,
4909,
399,
1722,
628,
198,
7804,
4242,
2,
198,
2235,
5045,
44709,
22492,
198,
7804,
4242,
2,
198,
198,
11748,
7308,
13,
1102,
1851,
198,
2235,
284,
5045,
276,
1045,
25,
11315,
21032,
532,
1464,
2499,
198,
8818,
10385,
7,
3712,
6030,
90,
14967,
276,
1045,
5512,
256,
77,
3712,
14967,
44709,
8,
198,
220,
220,
220,
1441,
5045,
276,
1045,
7,
34106,
13,
12786,
11,
256,
77,
13,
312,
87,
8,
198,
437,
198,
198,
2235,
284,
5045,
368,
265,
81,
25,
11315,
44890,
532,
10143,
329,
399,
1722,
198,
8818,
10385,
7,
3712,
6030,
90,
14967,
368,
265,
81,
5512,
256,
77,
3712,
14967,
44709,
11,
42721,
4535,
796,
3991,
8,
198,
220,
220,
220,
611,
42721,
4535,
198,
220,
220,
220,
220,
220,
220,
220,
256,
76,
796,
299,
1670,
7,
34106,
8,
198,
220,
220,
220,
220,
220,
220,
220,
256,
76,
796,
5045,
368,
265,
81,
7,
17209,
13,
12786,
11,
256,
76,
13,
312,
87,
8,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
256,
76,
796,
5045,
368,
265,
81,
7,
34106,
13,
12786,
11,
256,
77,
13,
312,
87,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
256,
76,
198,
437,
198,
198,
7804,
4242,
2235,
198,
2235,
5045,
368,
265,
81,
22492,
198,
7804,
4242,
2235,
198,
198,
2235,
284,
5045,
276,
1045,
25,
11315,
21032,
532,
1464,
2499,
198,
8818,
10385,
7,
3712,
6030,
90,
14967,
276,
1045,
5512,
256,
76,
3712,
14967,
368,
265,
81,
8,
198,
220,
220,
220,
5045,
276,
1045,
7,
17209,
13,
12786,
11,
256,
76,
13,
312,
87,
8,
198,
437,
198,
198,
2235,
284,
5045,
44709,
25,
11315,
21032,
532,
1464,
2499,
198,
8818,
10385,
7,
3712,
6030,
90,
14967,
44709,
5512,
256,
76,
3712,
14967,
368,
265,
81,
8,
198,
220,
220,
220,
5045,
44709,
7,
17209,
13,
12786,
11,
256,
76,
13,
312,
87,
8,
198,
437,
628,
198,
7804,
4242,
2235,
198,
2235,
5045,
276,
1045,
22492,
198,
7804,
4242,
2235,
198,
198,
2235,
284,
5045,
44709,
25,
11315,
44890,
532,
10143,
329,
1729,
12,
77,
39223,
3815,
198,
8818,
10385,
7,
3712,
6030,
90,
14967,
44709,
5512,
41560,
3712,
14967,
276,
1045,
8,
198,
220,
220,
220,
5045,
44709,
7,
8671,
13,
12786,
11,
41560,
13,
312,
87,
8,
198,
437,
198,
198,
2235,
284,
5045,
368,
265,
81,
25,
11315,
44890,
532,
10143,
329,
399,
1722,
198,
8818,
10385,
7,
3712,
6030,
90,
14967,
368,
265,
81,
5512,
41560,
3712,
14967,
276,
1045,
8,
198,
220,
220,
220,
5045,
368,
265,
81,
7,
8671,
13,
12786,
11,
41560,
13,
312,
87,
8,
198,
437,
198,
198,
14468,
198,
2235,
6060,
35439,
22492,
198,
14468,
198,
198,
2235,
10385,
6060,
19778,
351,
9667,
5721,
357,
292,
10903,
8,
284,
3862,
6601,
2134,
220,
198,
198,
8818,
10385,
7,
3712,
6030,
90,
23839,
14967,
276,
1045,
5512,
47764,
3712,
6601,
19778,
8,
198,
220,
220,
220,
22492,
1332,
611,
617,
5721,
1541,
318,
286,
2099,
7536,
198,
220,
220,
220,
611,
597,
7,
417,
19199,
7,
7568,
8,
764,
855,
7536,
8,
198,
220,
220,
220,
220,
220,
220,
220,
22492,
1011,
717,
1609,
870,
5721,
198,
220,
220,
220,
220,
220,
220,
220,
3128,
5216,
796,
1064,
7,
417,
19199,
7,
7568,
8,
764,
855,
7536,
38381,
16,
60,
198,
220,
220,
220,
220,
220,
220,
220,
4686,
87,
796,
10385,
7,
19182,
11,
47764,
58,
4475,
5216,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
12233,
0,
7,
7568,
11,
685,
4475,
5216,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
2073,
22492,
1064,
5721,
326,
3994,
9667,
355,
10903,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
1064,
15180,
326,
423,
587,
44267,
355,
4285,
654,
416,
1100,
11487,
198,
220,
220,
220,
220,
220,
220,
220,
951,
62,
1462,
62,
9288,
796,
15690,
7,
13940,
23650,
11,
657,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
299,
5216,
82,
796,
2546,
7,
7568,
11,
362,
8,
198,
220,
220,
220,
220,
220,
220,
220,
329,
21065,
28,
16,
25,
77,
5216,
82,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
318,
64,
7,
7568,
58,
16,
11,
21065,
4357,
10903,
19427,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
4033,
62,
1462,
62,
9288,
11,
3891,
7,
7568,
38381,
4178,
60,
2599,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2147,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
1332,
1123,
5721,
338,
1366,
284,
766,
611,
16092,
8079,
481,
21136,
340,
198,
220,
220,
220,
220,
220,
220,
220,
951,
62,
5562,
62,
6603,
796,
15690,
7,
13940,
23650,
11,
657,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
329,
951,
3672,
287,
951,
62,
1462,
62,
9288,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
288,
796,
2872,
7,
81,
17912,
22831,
59,
82,
91,
11139,
91,
8183,
1600,
47764,
58,
16,
11,
951,
3672,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
288,
5145,
855,
2147,
30,
357,
5657,
796,
6626,
7,
7568,
58,
16,
11,
951,
3672,
4357,
288,
13,
15699,
8,
2599,
357,
5657,
796,
685,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
4129,
7,
5657,
8,
6624,
513,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
4033,
62,
5562,
62,
6603,
11,
951,
3672,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
21136,
717,
5721,
326,
8318,
262,
16092,
8079,
40364,
1332,
198,
220,
220,
220,
220,
220,
220,
220,
4686,
87,
796,
7536,
58,
10430,
7,
67,
8,
329,
288,
287,
47764,
58,
4033,
62,
5562,
62,
6603,
58,
16,
11907,
60,
1303,
1231,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
7536,
340,
561,
2038,
442,
74,
7390,
87,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
287,
23772,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
12233,
0,
7,
7568,
11,
685,
4033,
62,
5562,
62,
6603,
58,
16,
11907,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
220,
198,
220,
220,
220,
22492,
1949,
1771,
6060,
19778,
11414,
850,
19199,
198,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
41560,
796,
5045,
368,
265,
81,
7,
7568,
11,
4686,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
41560,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
4929,
198,
220,
220,
220,
220,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
41560,
796,
5045,
44709,
7,
7568,
11,
4686,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1441,
41560,
198,
220,
220,
220,
220,
220,
220,
220,
4929,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
41560,
796,
5045,
276,
1045,
7,
7568,
11,
4686,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1441,
41560,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1441,
41560,
198,
437,
628,
198,
14468,
198,
2235,
3862,
3163,
20477,
22492,
198,
14468,
198,
198,
8818,
10385,
7,
3712,
6030,
90,
23839,
14967,
276,
1045,
5512,
20486,
3712,
7575,
19182,
8,
628,
220,
220,
220,
3891,
1722,
13940,
2022,
10220,
796,
685,
6601,
35439,
13,
738,
7483,
7,
7402,
8,
329,
299,
321,
287,
20486,
13,
4033,
14933,
60,
198,
220,
220,
220,
220,
198,
220,
220,
220,
47764,
796,
36664,
6601,
19778,
7,
8326,
13,
27160,
11,
3891,
1722,
13940,
2022,
10220,
8,
198,
220,
220,
220,
4686,
87,
796,
20486,
13,
16514,
27823,
628,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
41560,
796,
5045,
368,
265,
81,
7,
7568,
11,
4686,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
41560,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
4929,
198,
220,
220,
220,
220,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
41560,
796,
5045,
44709,
7,
7568,
11,
4686,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1441,
41560,
198,
220,
220,
220,
220,
220,
220,
220,
4929,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
41560,
796,
5045,
276,
1045,
7,
7568,
11,
4686,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1441,
41560,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
41560,
198,
437,
198,
198,
8818,
10385,
7,
3712,
6030,
90,
7575,
19182,
5512,
256,
77,
3712,
23839,
14967,
44709,
8,
198,
220,
220,
220,
288,
1381,
796,
4686,
87,
7,
34106,
8,
198,
220,
220,
220,
299,
4105,
796,
41002,
23,
10100,
58,
8841,
7,
14933,
7,
34106,
38381,
4178,
12962,
329,
21065,
796,
352,
25,
7857,
7,
34106,
11,
362,
15437,
198,
220,
220,
220,
1441,
3862,
19182,
7,
67,
1381,
11,
355,
3163,
81,
7,
34106,
11,
48436,
2414,
11,
11013,
45,
828,
299,
4105,
8,
198,
437,
198
] | 2.176519 | 2,436 |
# SINGLE NODE/CORE VERSION
import Pkg; Pkg.instantiate()
include("/users/yh31/scratch/projects/gigaword_64k/src/gigaword_64k.jl")
const path_apw_dir = "/users/yh31/scratch/datasets/entity_linking/raw_data/gigaword/giga5/data/apw_eng"
const path_intermed_data = "/users/yh31/scratch/datasets/entity_linking/raw_data/gigaword/giga_topk/intermediate_files"
const path_intermed_apw = joinpath(path_intermed_data, "apw")
process_part_of_tree(path_apw_dir, path_intermed_apw, 4)
| [
2,
311,
2751,
2538,
399,
16820,
14,
34,
6965,
44156,
2849,
198,
198,
11748,
350,
10025,
26,
350,
10025,
13,
8625,
415,
9386,
3419,
198,
198,
17256,
7203,
14,
18417,
14,
88,
71,
3132,
14,
1416,
36722,
14,
42068,
14,
70,
328,
707,
585,
62,
2414,
74,
14,
10677,
14,
70,
328,
707,
585,
62,
2414,
74,
13,
20362,
4943,
198,
198,
9979,
3108,
62,
499,
86,
62,
15908,
796,
12813,
18417,
14,
88,
71,
3132,
14,
1416,
36722,
14,
19608,
292,
1039,
14,
26858,
62,
75,
8040,
14,
1831,
62,
7890,
14,
70,
328,
707,
585,
14,
70,
13827,
20,
14,
7890,
14,
499,
86,
62,
1516,
1,
198,
198,
9979,
3108,
62,
3849,
1150,
62,
7890,
796,
12813,
18417,
14,
88,
71,
3132,
14,
1416,
36722,
14,
19608,
292,
1039,
14,
26858,
62,
75,
8040,
14,
1831,
62,
7890,
14,
70,
328,
707,
585,
14,
70,
13827,
62,
4852,
74,
14,
3849,
13857,
62,
16624,
1,
198,
9979,
3108,
62,
3849,
1150,
62,
499,
86,
796,
4654,
6978,
7,
6978,
62,
3849,
1150,
62,
7890,
11,
366,
499,
86,
4943,
628,
198,
198,
14681,
62,
3911,
62,
1659,
62,
21048,
7,
6978,
62,
499,
86,
62,
15908,
11,
3108,
62,
3849,
1150,
62,
499,
86,
11,
604,
8,
198
] | 2.274882 | 211 |
# fundamental types (expanded below)
# nodes in the AST that describe the grammar. all Matcher instances must be
# mutable and have an attribute
# name::Symbol
# which is set automatically to the matcher type by the constructor.
# (re-set to a more useful type inside with_names() - see names.jl)
abstract type Matcher end
abstract type Message end # data sent between trampoline and methods
abstract type State end # state associated with Matchers during evaluation
# used to configure the parser. all Config subtypes must have associated
# dispatch functions (see parser.jl), a parent() function, and have a
# constructor that takes the source as first argument and additional arguments
# as keywords. the type of the source is exposed and if it's a subclass of
# string then the iterator is assumed to be a simple integer index.
abstract type Config{S,I} end
# important notes on mutability / hash / equality
# 1 - immutable types in julia are not "just" immutable. they are
# effectively values - they are passed by value. so do not use
# "immutable" just because the data should not change. think about
# details.
# 2 - immutable types have an automatic equality and hash based on
# content (which is copied). mutable types have an automatic equality
# and hash based on address. so default hash and equality for
# immutable types that contain mutable types, and for mutable types,
# may not be what is required.
# 3 - caching within the parser REQUIRES that both Matcher and State
# instances have 'useful' equality and hash values.
# 4 - for matchers, which are created when the grammar is defined, and
# then unchanged, the default hash and equality are likely just fine,
# even for mutable objects (in fact, mutable may be slightly faster
# since equality is just a comparison of an Int64 address,
# presumably).
# 5 - for states, which often includes mutable result objects, more
# care is needed:
# 5a - whether or not State instances are mutable or immutable, they,
# and their contents, must not change during matching. so all arrays,
# for example, must be copied when new instances are created with
# different values.
# 5b - structurally identical states must be equal, and hash equally.
# this is critical for efficient caching. so it it likely that
# custom hash and equality methods will be needed (see above and
# auto.jl).
# defaults for mismatching types and types with no content
==(a::Matcher, b::Matcher) = false
==(a::T, b::T) where {T<:Matcher} = true
==(a::State, b::State) = false
==(a::T, b::T) where {T<:State} = true
# use an array to handle empty values in a natural way
const Value = Vector{Any}
const EMPTY = Any[]
function flatten(x::Array{Value,1}) where {Value}
y::Value = vcat(x...)
return y
end
# Message sub-types
# use mutable types here since they are packed and unpacked often
# parent and parent_state are popped from the stack. a call is made to
# success(config, parent, parent_state, child_state, iter, result)
struct Success{CS<:State,I}<:Message
child_state::CS # parent to store, passed in next call for backtracking
iter::I # advanced as appropriate
result::Value # possibly empty
end
# parent and parent_state are popped from the stack. a call is made to
# failure(config, parent, parent_state)
struct Failure<:Message end
const FAILURE = Failure()
# parent and parent_state are pushed to the stack. a call is made to
# execute(config, child, child_state, iter)
struct Execute{I}<:Message
parent::Matcher # stored by trampoline, added to response
parent_state::State # stored by trampoline, added to response
child::Matcher # the matcher to evaluate
child_state::State # needed by for evaluation (was stored by parent)
iter::I
end
# State sub-types
# use immutable types because these are simple, magic values
# the state used on first call
struct Clean<:State end
const CLEAN = Clean()
# the state used when no further calls should be made
struct Dirty<:State end
const DIRTY = Dirty()
# other stuff
# user-generated errors (ie bad input, etc).
# internal errors in the library (bugs) may raise Error
struct ParserException<:Exception
msg
end
# this cannot be cached (thrown by hash())
struct CacheException<:Exception end
# this is equivalent to a matcher returning Failure. used when source
# information is not available.
abstract type FailureException<:Exception end
const Applicable = Union{Function, DataType}
| [
198,
198,
2,
7531,
3858,
357,
11201,
12249,
2174,
8,
198,
198,
2,
13760,
287,
262,
29273,
326,
6901,
262,
23491,
13,
220,
477,
6550,
2044,
10245,
1276,
307,
198,
2,
4517,
540,
290,
423,
281,
11688,
198,
2,
220,
1438,
3712,
13940,
23650,
198,
2,
543,
318,
900,
6338,
284,
262,
2603,
2044,
2099,
416,
262,
23772,
13,
198,
2,
357,
260,
12,
2617,
284,
257,
517,
4465,
2099,
2641,
351,
62,
14933,
3419,
532,
766,
3891,
13,
20362,
8,
198,
397,
8709,
2099,
6550,
2044,
886,
198,
198,
397,
8709,
2099,
16000,
886,
220,
220,
1303,
1366,
1908,
1022,
491,
696,
14453,
290,
5050,
198,
397,
8709,
2099,
1812,
886,
220,
220,
220,
1303,
1181,
3917,
351,
6550,
3533,
1141,
12660,
198,
198,
2,
973,
284,
17425,
262,
30751,
13,
220,
477,
17056,
850,
19199,
1276,
423,
3917,
198,
2,
27965,
5499,
357,
3826,
30751,
13,
20362,
828,
257,
2560,
3419,
2163,
11,
290,
423,
257,
198,
2,
23772,
326,
2753,
262,
2723,
355,
717,
4578,
290,
3224,
7159,
198,
2,
355,
26286,
13,
220,
262,
2099,
286,
262,
2723,
318,
7362,
290,
611,
340,
338,
257,
47611,
286,
198,
2,
4731,
788,
262,
41313,
318,
9672,
284,
307,
257,
2829,
18253,
6376,
13,
198,
397,
8709,
2099,
17056,
90,
50,
11,
40,
92,
886,
628,
198,
2,
1593,
4710,
319,
4517,
1799,
1220,
12234,
1220,
10537,
198,
198,
2,
352,
532,
40139,
3858,
287,
474,
43640,
389,
407,
366,
3137,
1,
40139,
13,
220,
484,
389,
198,
2,
6840,
3815,
532,
484,
389,
3804,
416,
1988,
13,
220,
523,
466,
407,
779,
198,
2,
366,
8608,
18187,
1,
655,
780,
262,
1366,
815,
407,
1487,
13,
220,
892,
546,
198,
2,
3307,
13,
198,
198,
2,
362,
532,
40139,
3858,
423,
281,
11353,
10537,
290,
12234,
1912,
319,
198,
2,
2695,
357,
4758,
318,
18984,
737,
220,
4517,
540,
3858,
423,
281,
11353,
10537,
198,
2,
290,
12234,
1912,
319,
2209,
13,
220,
523,
4277,
12234,
290,
10537,
329,
198,
2,
40139,
3858,
326,
3994,
4517,
540,
3858,
11,
290,
329,
4517,
540,
3858,
11,
198,
2,
743,
407,
307,
644,
318,
2672,
13,
198,
198,
2,
513,
532,
40918,
1626,
262,
30751,
4526,
10917,
4663,
1546,
326,
1111,
6550,
2044,
290,
1812,
198,
2,
10245,
423,
705,
1904,
913,
6,
10537,
290,
12234,
3815,
13,
198,
198,
2,
604,
532,
329,
2603,
3533,
11,
543,
389,
2727,
618,
262,
23491,
318,
5447,
11,
290,
198,
2,
788,
21588,
11,
262,
4277,
12234,
290,
10537,
389,
1884,
655,
3734,
11,
198,
2,
772,
329,
4517,
540,
5563,
357,
259,
1109,
11,
4517,
540,
743,
307,
4622,
5443,
198,
2,
1201,
10537,
318,
655,
257,
7208,
286,
281,
2558,
2414,
2209,
11,
198,
2,
14572,
737,
198,
198,
2,
642,
532,
329,
2585,
11,
543,
1690,
3407,
4517,
540,
1255,
5563,
11,
517,
198,
2,
1337,
318,
2622,
25,
198,
198,
2,
642,
64,
532,
1771,
393,
407,
1812,
10245,
389,
4517,
540,
393,
40139,
11,
484,
11,
198,
2,
290,
511,
10154,
11,
1276,
407,
1487,
1141,
12336,
13,
220,
523,
477,
26515,
11,
198,
2,
329,
1672,
11,
1276,
307,
18984,
618,
649,
10245,
389,
2727,
351,
198,
2,
1180,
3815,
13,
198,
198,
2,
642,
65,
532,
2878,
20221,
10411,
2585,
1276,
307,
4961,
11,
290,
12234,
8603,
13,
198,
2,
428,
318,
4688,
329,
6942,
40918,
13,
220,
523,
340,
340,
1884,
326,
198,
2,
2183,
12234,
290,
10537,
5050,
481,
307,
2622,
357,
3826,
2029,
290,
198,
2,
8295,
13,
20362,
737,
628,
198,
2,
26235,
329,
32691,
19775,
3858,
290,
3858,
351,
645,
2695,
198,
855,
7,
64,
3712,
19044,
2044,
11,
275,
3712,
19044,
2044,
8,
796,
3991,
198,
855,
7,
64,
3712,
51,
11,
275,
3712,
51,
8,
810,
1391,
51,
27,
25,
19044,
2044,
92,
796,
2081,
198,
855,
7,
64,
3712,
9012,
11,
275,
3712,
9012,
8,
796,
3991,
198,
855,
7,
64,
3712,
51,
11,
275,
3712,
51,
8,
810,
1391,
51,
27,
25,
9012,
92,
796,
2081,
628,
198,
2,
779,
281,
7177,
284,
5412,
6565,
3815,
287,
257,
3288,
835,
198,
198,
9979,
11052,
796,
20650,
90,
7149,
92,
198,
198,
9979,
38144,
9936,
796,
4377,
21737,
198,
198,
8818,
27172,
268,
7,
87,
3712,
19182,
90,
11395,
11,
16,
30072,
810,
1391,
11395,
92,
198,
220,
220,
220,
331,
3712,
11395,
796,
410,
9246,
7,
87,
23029,
198,
220,
220,
220,
1441,
331,
198,
437,
628,
628,
198,
2,
16000,
850,
12,
19199,
198,
198,
2,
779,
4517,
540,
3858,
994,
1201,
484,
389,
11856,
290,
8593,
6021,
1690,
198,
198,
2,
2560,
290,
2560,
62,
5219,
389,
22928,
422,
262,
8931,
13,
220,
257,
869,
318,
925,
284,
198,
2,
1943,
7,
11250,
11,
2560,
11,
2560,
62,
5219,
11,
1200,
62,
5219,
11,
11629,
11,
1255,
8,
198,
7249,
16282,
90,
7902,
27,
25,
9012,
11,
40,
92,
27,
25,
12837,
198,
220,
220,
220,
1200,
62,
5219,
3712,
7902,
220,
220,
1303,
2560,
284,
3650,
11,
3804,
287,
1306,
869,
329,
736,
36280,
198,
220,
220,
220,
11629,
3712,
40,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
6190,
355,
5035,
198,
220,
220,
220,
1255,
3712,
11395,
220,
220,
220,
220,
1303,
5457,
6565,
198,
437,
198,
198,
2,
2560,
290,
2560,
62,
5219,
389,
22928,
422,
262,
8931,
13,
220,
257,
869,
318,
925,
284,
198,
2,
5287,
7,
11250,
11,
2560,
11,
2560,
62,
5219,
8,
198,
7249,
25743,
27,
25,
12837,
886,
198,
9979,
9677,
4146,
11335,
796,
25743,
3419,
198,
198,
2,
2560,
290,
2560,
62,
5219,
389,
7121,
284,
262,
8931,
13,
220,
257,
869,
318,
925,
284,
198,
2,
12260,
7,
11250,
11,
1200,
11,
1200,
62,
5219,
11,
11629,
8,
198,
7249,
8393,
1133,
90,
40,
92,
27,
25,
12837,
198,
220,
220,
220,
2560,
3712,
19044,
2044,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
8574,
416,
491,
696,
14453,
11,
2087,
284,
2882,
198,
220,
220,
220,
2560,
62,
5219,
3712,
9012,
220,
1303,
8574,
416,
491,
696,
14453,
11,
2087,
284,
2882,
198,
220,
220,
220,
1200,
3712,
19044,
2044,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
262,
2603,
2044,
284,
13446,
198,
220,
220,
220,
1200,
62,
5219,
3712,
9012,
220,
220,
1303,
2622,
416,
329,
12660,
357,
9776,
8574,
416,
2560,
8,
198,
220,
220,
220,
11629,
3712,
40,
198,
437,
628,
198,
198,
2,
1812,
850,
12,
19199,
198,
198,
2,
779,
40139,
3858,
780,
777,
389,
2829,
11,
5536,
3815,
198,
198,
2,
262,
1181,
973,
319,
717,
869,
198,
7249,
5985,
27,
25,
9012,
886,
198,
9979,
30301,
1565,
796,
5985,
3419,
198,
198,
2,
262,
1181,
973,
618,
645,
2252,
3848,
815,
307,
925,
198,
7249,
32052,
27,
25,
9012,
886,
198,
9979,
360,
4663,
9936,
796,
32052,
3419,
628,
198,
198,
2,
584,
3404,
198,
198,
2,
2836,
12,
27568,
8563,
357,
494,
2089,
5128,
11,
3503,
737,
198,
2,
5387,
8563,
287,
262,
5888,
357,
32965,
8,
743,
5298,
13047,
198,
7249,
23042,
263,
16922,
27,
25,
16922,
198,
220,
220,
220,
31456,
198,
437,
198,
198,
2,
428,
2314,
307,
39986,
357,
400,
2053,
416,
12234,
28955,
198,
7249,
34088,
16922,
27,
25,
16922,
886,
198,
198,
2,
428,
318,
7548,
284,
257,
2603,
2044,
8024,
25743,
13,
220,
973,
618,
2723,
198,
2,
1321,
318,
407,
1695,
13,
198,
397,
8709,
2099,
25743,
16922,
27,
25,
16922,
886,
198,
198,
9979,
16806,
540,
796,
4479,
90,
22203,
11,
6060,
6030,
92,
198
] | 3.583797 | 1,259 |
import Base: ==
mutable struct Point{T<:Number}
x::T
y::T
end
==(p::Point, q::Point) = p.x == q.x && p.y == q.y
@enum Heading NORTH=1 EAST=2 SOUTH=3 WEST=4
mutable struct Robot
position::Point
heading::Heading
end
function Robot(position::Tuple{T, T}, heading::Heading) where T<:Number
Robot(Point(position...), heading)
end
heading(r::Robot) = r.heading
position(r::Robot) = r.position
function turn_right!(r::Robot)
r.heading = Heading(mod1(Int(r.heading) + 1, 4))
r
end
function turn_left!(r::Robot)
r.heading = Heading(mod1(Int(r.heading) - 1, 4))
r
end
function advance!(r::Robot)
if heading(r) == NORTH
r.position.y += 1
elseif heading(r) == SOUTH
r.position.y -= 1
elseif heading(r) == EAST
r.position.x += 1
else
r.position.x -= 1
end
r
end
function move!(r::Robot, instructions::AbstractString)
moves = Dict('A' => advance!, 'R' => turn_right!, 'L' => turn_left!)
for move in instructions
move in keys(moves) && moves[move](r)
end
r
end
| [
11748,
7308,
25,
6624,
198,
198,
76,
18187,
2878,
6252,
90,
51,
27,
25,
15057,
92,
198,
220,
220,
220,
2124,
3712,
51,
198,
220,
220,
220,
331,
3712,
51,
198,
437,
198,
198,
855,
7,
79,
3712,
12727,
11,
10662,
3712,
12727,
8,
796,
279,
13,
87,
6624,
10662,
13,
87,
11405,
279,
13,
88,
6624,
10662,
13,
88,
198,
198,
31,
44709,
679,
4980,
25273,
4221,
28,
16,
412,
11262,
28,
17,
30065,
4221,
28,
18,
370,
6465,
28,
19,
198,
198,
76,
18187,
2878,
16071,
198,
220,
220,
220,
2292,
3712,
12727,
198,
220,
220,
220,
9087,
3712,
13847,
278,
198,
437,
198,
198,
8818,
16071,
7,
9150,
3712,
51,
29291,
90,
51,
11,
309,
5512,
9087,
3712,
13847,
278,
8,
810,
309,
27,
25,
15057,
198,
220,
220,
220,
16071,
7,
12727,
7,
9150,
986,
828,
9087,
8,
198,
437,
198,
198,
33878,
7,
81,
3712,
14350,
313,
8,
796,
374,
13,
33878,
198,
9150,
7,
81,
3712,
14350,
313,
8,
796,
374,
13,
9150,
198,
198,
8818,
1210,
62,
3506,
0,
7,
81,
3712,
14350,
313,
8,
198,
220,
220,
220,
374,
13,
33878,
796,
679,
4980,
7,
4666,
16,
7,
5317,
7,
81,
13,
33878,
8,
1343,
352,
11,
604,
4008,
198,
220,
220,
220,
374,
198,
437,
198,
198,
8818,
1210,
62,
9464,
0,
7,
81,
3712,
14350,
313,
8,
198,
220,
220,
220,
374,
13,
33878,
796,
679,
4980,
7,
4666,
16,
7,
5317,
7,
81,
13,
33878,
8,
532,
352,
11,
604,
4008,
198,
220,
220,
220,
374,
198,
437,
198,
198,
8818,
5963,
0,
7,
81,
3712,
14350,
313,
8,
198,
220,
220,
220,
611,
9087,
7,
81,
8,
6624,
25273,
4221,
198,
220,
220,
220,
220,
220,
220,
220,
374,
13,
9150,
13,
88,
15853,
352,
198,
220,
220,
220,
2073,
361,
9087,
7,
81,
8,
6624,
30065,
4221,
198,
220,
220,
220,
220,
220,
220,
220,
374,
13,
9150,
13,
88,
48185,
352,
198,
220,
220,
220,
2073,
361,
9087,
7,
81,
8,
6624,
412,
11262,
198,
220,
220,
220,
220,
220,
220,
220,
374,
13,
9150,
13,
87,
15853,
352,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
374,
13,
9150,
13,
87,
48185,
352,
198,
220,
220,
220,
886,
198,
220,
220,
220,
374,
198,
437,
198,
198,
8818,
1445,
0,
7,
81,
3712,
14350,
313,
11,
7729,
3712,
23839,
10100,
8,
198,
220,
220,
220,
6100,
796,
360,
713,
10786,
32,
6,
5218,
5963,
28265,
705,
49,
6,
5218,
1210,
62,
3506,
28265,
705,
43,
6,
5218,
1210,
62,
9464,
8133,
198,
220,
220,
220,
329,
1445,
287,
7729,
198,
220,
220,
220,
220,
220,
220,
220,
1445,
287,
8251,
7,
76,
5241,
8,
11405,
6100,
58,
21084,
16151,
81,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
374,
198,
437,
198
] | 2.261603 | 474 |
# ================================================================================================
# Main API
using FixedEffectModels
@reexport using FixedEffectModels
import StatsModels: width, apply_schema
anova(trms::Vararg{TableRegressionModel{<: FixedEffectModel}};
test::Type{<: GoodnessOfFit} = FTest,
kwargs...) =
anova(test, trms...; kwargs...)
# ================================================================================================
# ANOVA by F test
function anova(::Type{FTest},
trm::TableRegressionModel{<: FixedEffectModel};
type::Int = 1, kwargs...)
type == 2 && throw(ArgumentError("Type 2 anova is not implemented"))
type in [1, 2, 3] || throw(ArgumentError("Invalid type"))
assign = trm.mm.assign
df = dof(assign)
filter!(>(0), df)
# May exist some floating point error from dof_residual
push!(df, round(Int, dof_residual(trm)))
df = tuple(df...)
if type in [1, 3]
# vcov methods
varβ = vcov(trm)
β = trm.model.coef
if type == 1
fs = abs2.(cholesky(Hermitian(inv(varβ))).U * β)
offset = first(assign) - 1
fstat = ntuple(last(assign) - offset) do fix
sum(fs[findall(==(fix + offset), assign)]) / df[fix]
end
else
# calculate block by block
offset = first(assign) - 1
fstat = ntuple(last(assign) - offset) do fix
select = findall(==(fix + offset), assign)
β[select]' * (varβ[select, select] \ β[select]) / df[fix]
end
end
σ² = rss(trm.model) / last(df)
devs = (fstat .* σ²..., σ²) .* df
end
pvalue = (ccdf.(FDist.(df[1:end - 1], last(df)), abs.(fstat))..., NaN)
AnovaResult{FTest}(trm, type, df, devs, (fstat..., NaN), pvalue, NamedTuple())
end
# =================================================================================================================
# Nested models
function anova(::Type{FTest},
trms::Vararg{TableRegressionModel{<: FixedEffectModel}};
check::Bool = true,
isnested::Bool = false)
df = dof.(trms)
ord = sortperm(collect(df))
df = df[ord]
trms = trms[ord]
# check comparable and nested
check && @warn "Could not check whether models are nested: results may not be meaningful"
Δdf = _diff(df)
# May exist some floating point error from dof_residual
dfr = round.(Int, dof_residual.(trms))
dev = ntuple(length(trms)) do i
trms[i].model.rss
end
msr = _diffn(dev) ./Δdf
σ² = last(dev) / last(dfr)
fstat = msr ./ σ²
pval = map(zip(Δdf, dfr[2:end], fstat)) do (dof, dofr, fs)
fs > 0 ? ccdf(FDist(dof, dofr), fs) : NaN
end
AnovaResult{FTest}(trms, 1, df, dev, (NaN, fstat...), (NaN, pval...), NamedTuple())
end
"""
lfe(formula::FormulaTerm, df, vcov::CovarianceEstimator = Vcov.simple(); kwargs...)
Fit a `FixedEffectModel` and wrap it into `TableRegressionModel`.
!!! warn
This function currently does not perform well. It re-compiles everytime; may be due to `@nonspecialize` for parameters of `reg`.
"""
lfe(formula::FormulaTerm, df, vcov::CovarianceEstimator = Vcov.simple(); kwargs...) =
to_trm(reg(df, formula, vcov; kwargs...), df)
"""
to_trm(model, df)
Wrap fitted `FixedEffectModel` into `TableRegressionModel`.
"""
function to_trm(model::FixedEffectModel, df)
f = model.formula
has_fe_intercept = any(fe_intercept(f))
rhs = vectorize(f.rhs)
f = isa(first(rhs), ConstantTerm) ? f : FormulaTerm(f.lhs, (ConstantTerm(1), rhs...))
s = schema(f, df, model.contrasts)
f = apply_schema(f, s, FixedEffectModel, has_fe_intercept)
mf = ModelFrame(f, s, Tables.columntable(df[!, getproperty.(keys(s), :sym)]), FixedEffectModel)
# Fake modelmatrix
assign = asgn(f)
has_fe_intercept && popfirst!(assign)
mm = ModelMatrix(ones(Float64, 1, 1), assign)
TableRegressionModel(model, mf, mm)
end
# =================================================================================================================================
# Fit new models
"""
anova_lfe(f::FormulaTerm, df, vcov::CovarianceEstimator = Vcov.simple();
test::Type{<: GoodnessOfFit} = FTest, <keyword arguments>)
anova_lfe(test::Type{<: GoodnessOfFit}, f::FormulaTerm, df, vcov::CovarianceEstimator = Vcov.simple(); <keyword arguments>)
ANOVA for fixed-effect linear regression.
* `vcov`: estimator of covariance matrix.
* `type`: type of anova.
`anova_lfe` generate a `TableRegressionModel{<: FixedEffectModel}`.
"""
anova_lfe(f::FormulaTerm, df, vcov::CovarianceEstimator = Vcov.simple();
test::Type{<: GoodnessOfFit} = FTest,
kwargs...)=
anova(test, FixedEffectModel, f, df, vcov; kwargs...)
anova_lfe(test::Type{<: GoodnessOfFit}, f::FormulaTerm, df, vcov::CovarianceEstimator = Vcov.simple(); kwargs...) =
anova(test, FixedEffectModel, f, df, vcov; kwargs...)
function anova(test::Type{<: GoodnessOfFit}, ::Type{FixedEffectModel}, f::FormulaTerm, df, vcov::CovarianceEstimator = Vcov.simple();
type::Int = 1,
kwargs...)
trm = to_trm(reg(df, f, vcov; kwargs...), df)
anova(test, trm; type)
end
| [
2,
38093,
4770,
25609,
18604,
198,
2,
8774,
7824,
198,
198,
3500,
10832,
18610,
5841,
1424,
198,
31,
631,
87,
634,
1262,
10832,
18610,
5841,
1424,
198,
11748,
20595,
5841,
1424,
25,
9647,
11,
4174,
62,
15952,
2611,
198,
198,
40993,
7,
2213,
907,
3712,
19852,
853,
90,
10962,
8081,
2234,
17633,
90,
27,
25,
10832,
18610,
17633,
11709,
26,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1332,
3712,
6030,
90,
27,
25,
4599,
1108,
5189,
31805,
92,
796,
376,
14402,
11,
198,
220,
220,
220,
220,
220,
220,
220,
479,
86,
22046,
23029,
796,
220,
198,
220,
220,
220,
281,
10071,
7,
9288,
11,
491,
907,
986,
26,
479,
86,
22046,
23029,
198,
198,
2,
38093,
4770,
25609,
18604,
198,
2,
3537,
41576,
416,
376,
1332,
198,
198,
8818,
281,
10071,
7,
3712,
6030,
90,
9792,
395,
5512,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
491,
76,
3712,
10962,
8081,
2234,
17633,
90,
27,
25,
10832,
18610,
17633,
19629,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2099,
3712,
5317,
796,
352,
11,
479,
86,
22046,
23029,
628,
220,
220,
220,
2099,
6624,
362,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
11405,
3714,
7,
28100,
1713,
12331,
7203,
6030,
362,
281,
10071,
318,
407,
9177,
48774,
198,
220,
220,
220,
2099,
287,
685,
16,
11,
362,
11,
513,
60,
220,
220,
8614,
3714,
7,
28100,
1713,
12331,
7203,
44651,
2099,
48774,
198,
220,
220,
220,
8333,
796,
491,
76,
13,
3020,
13,
562,
570,
198,
220,
220,
220,
47764,
796,
466,
69,
7,
562,
570,
8,
198,
220,
220,
220,
8106,
0,
7,
33994,
15,
828,
47764,
8,
198,
220,
220,
220,
1303,
1737,
2152,
617,
12462,
966,
4049,
422,
466,
69,
62,
411,
312,
723,
198,
220,
220,
220,
4574,
0,
7,
7568,
11,
2835,
7,
5317,
11,
466,
69,
62,
411,
312,
723,
7,
2213,
76,
22305,
198,
220,
220,
220,
47764,
796,
46545,
7,
7568,
23029,
198,
220,
220,
220,
611,
2099,
287,
685,
16,
11,
513,
60,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
410,
66,
709,
5050,
198,
220,
220,
220,
220,
220,
220,
220,
1401,
26638,
796,
410,
66,
709,
7,
2213,
76,
8,
198,
220,
220,
220,
220,
220,
220,
220,
27169,
796,
491,
76,
13,
19849,
13,
1073,
891,
198,
220,
220,
220,
220,
220,
220,
220,
611,
2099,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
43458,
796,
2352,
17,
12195,
354,
4316,
2584,
7,
9360,
2781,
666,
7,
16340,
7,
7785,
26638,
4008,
737,
52,
1635,
27169,
8,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
11677,
796,
717,
7,
562,
570,
8,
532,
352,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
277,
14269,
796,
299,
83,
29291,
7,
12957,
7,
562,
570,
8,
532,
11677,
8,
466,
4259,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2160,
7,
9501,
58,
19796,
439,
7,
855,
7,
13049,
1343,
11677,
828,
8333,
8,
12962,
1220,
47764,
58,
13049,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
15284,
2512,
416,
2512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
11677,
796,
717,
7,
562,
570,
8,
532,
352,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
277,
14269,
796,
299,
83,
29291,
7,
12957,
7,
562,
570,
8,
532,
11677,
8,
466,
4259,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2922,
796,
1064,
439,
7,
855,
7,
13049,
1343,
11677,
828,
8333,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
27169,
58,
19738,
49946,
1635,
357,
7785,
26638,
58,
19738,
11,
2922,
60,
3467,
27169,
58,
19738,
12962,
1220,
47764,
58,
13049,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
18074,
225,
31185,
796,
374,
824,
7,
2213,
76,
13,
19849,
8,
1220,
938,
7,
7568,
8,
198,
220,
220,
220,
220,
220,
220,
220,
41470,
796,
357,
69,
14269,
764,
9,
18074,
225,
31185,
986,
11,
18074,
225,
31185,
8,
764,
9,
47764,
198,
220,
220,
220,
886,
198,
220,
220,
220,
279,
8367,
796,
357,
535,
7568,
12195,
37,
20344,
12195,
7568,
58,
16,
25,
437,
532,
352,
4357,
938,
7,
7568,
36911,
2352,
12195,
69,
14269,
4008,
986,
11,
11013,
45,
8,
198,
220,
220,
220,
1052,
10071,
23004,
90,
9792,
395,
92,
7,
2213,
76,
11,
2099,
11,
47764,
11,
41470,
11,
357,
69,
14269,
986,
11,
11013,
45,
828,
279,
8367,
11,
34441,
51,
29291,
28955,
198,
437,
198,
198,
2,
38093,
10052,
4770,
198,
2,
399,
7287,
4981,
220,
198,
198,
8818,
281,
10071,
7,
3712,
6030,
90,
9792,
395,
5512,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
491,
907,
3712,
19852,
853,
90,
10962,
8081,
2234,
17633,
90,
27,
25,
10832,
18610,
17633,
11709,
26,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2198,
3712,
33,
970,
796,
2081,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2125,
7287,
3712,
33,
970,
796,
3991,
8,
628,
220,
220,
220,
47764,
796,
466,
69,
12195,
2213,
907,
8,
198,
220,
220,
220,
2760,
796,
3297,
16321,
7,
33327,
7,
7568,
4008,
198,
220,
220,
220,
47764,
796,
47764,
58,
585,
60,
198,
220,
220,
220,
491,
907,
796,
491,
907,
58,
585,
60,
628,
220,
220,
220,
1303,
2198,
13975,
290,
28376,
198,
220,
220,
220,
2198,
11405,
2488,
40539,
366,
23722,
407,
2198,
1771,
4981,
389,
28376,
25,
2482,
743,
407,
307,
11570,
1,
628,
220,
220,
220,
37455,
7568,
796,
4808,
26069,
7,
7568,
8,
198,
220,
220,
220,
1303,
1737,
2152,
617,
12462,
966,
4049,
422,
466,
69,
62,
411,
312,
723,
198,
220,
220,
220,
288,
8310,
796,
2835,
12195,
5317,
11,
466,
69,
62,
411,
312,
723,
12195,
2213,
907,
4008,
198,
220,
220,
220,
1614,
796,
299,
83,
29291,
7,
13664,
7,
2213,
907,
4008,
466,
1312,
220,
198,
220,
220,
220,
220,
220,
220,
220,
491,
907,
58,
72,
4083,
19849,
13,
42216,
198,
220,
220,
220,
886,
198,
220,
220,
220,
13845,
81,
796,
4808,
26069,
77,
7,
7959,
8,
24457,
138,
242,
7568,
198,
220,
220,
220,
18074,
225,
31185,
796,
938,
7,
7959,
8,
1220,
938,
7,
7568,
81,
8,
198,
220,
220,
220,
277,
14269,
796,
13845,
81,
24457,
18074,
225,
31185,
198,
220,
220,
220,
279,
2100,
796,
3975,
7,
13344,
7,
138,
242,
7568,
11,
288,
8310,
58,
17,
25,
437,
4357,
277,
14269,
4008,
466,
357,
67,
1659,
11,
466,
8310,
11,
43458,
8,
198,
220,
220,
220,
220,
220,
220,
220,
43458,
1875,
657,
5633,
36624,
7568,
7,
37,
20344,
7,
67,
1659,
11,
466,
8310,
828,
43458,
8,
1058,
11013,
45,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1052,
10071,
23004,
90,
9792,
395,
92,
7,
2213,
907,
11,
352,
11,
47764,
11,
1614,
11,
357,
26705,
45,
11,
277,
14269,
986,
828,
357,
26705,
45,
11,
279,
2100,
986,
828,
34441,
51,
29291,
28955,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
300,
5036,
7,
687,
4712,
3712,
8479,
4712,
40596,
11,
47764,
11,
410,
66,
709,
3712,
34,
709,
2743,
590,
22362,
320,
1352,
796,
569,
66,
709,
13,
36439,
9783,
479,
86,
22046,
23029,
198,
198,
31805,
257,
4600,
13715,
18610,
17633,
63,
290,
14441,
340,
656,
4600,
10962,
8081,
2234,
17633,
44646,
220,
198,
10185,
9828,
198,
220,
220,
220,
770,
2163,
3058,
857,
407,
1620,
880,
13,
632,
302,
12,
5589,
2915,
790,
2435,
26,
743,
307,
2233,
284,
4600,
31,
77,
684,
431,
2413,
1096,
63,
329,
10007,
286,
4600,
2301,
44646,
198,
37811,
198,
1652,
68,
7,
687,
4712,
3712,
8479,
4712,
40596,
11,
47764,
11,
410,
66,
709,
3712,
34,
709,
2743,
590,
22362,
320,
1352,
796,
569,
66,
709,
13,
36439,
9783,
479,
86,
22046,
23029,
796,
220,
198,
220,
220,
220,
284,
62,
2213,
76,
7,
2301,
7,
7568,
11,
10451,
11,
410,
66,
709,
26,
479,
86,
22046,
986,
828,
47764,
8,
198,
198,
37811,
198,
220,
220,
220,
284,
62,
2213,
76,
7,
19849,
11,
47764,
8,
198,
198,
54,
2416,
18235,
4600,
13715,
18610,
17633,
63,
656,
4600,
10962,
8081,
2234,
17633,
44646,
198,
37811,
198,
8818,
284,
62,
2213,
76,
7,
19849,
3712,
13715,
18610,
17633,
11,
47764,
8,
198,
220,
220,
220,
277,
796,
2746,
13,
687,
4712,
198,
220,
220,
220,
468,
62,
5036,
62,
3849,
984,
796,
597,
7,
5036,
62,
3849,
984,
7,
69,
4008,
198,
220,
220,
220,
9529,
82,
796,
15879,
1096,
7,
69,
13,
81,
11994,
8,
198,
220,
220,
220,
277,
796,
318,
64,
7,
11085,
7,
81,
11994,
828,
20217,
40596,
8,
5633,
277,
1058,
19639,
40596,
7,
69,
13,
75,
11994,
11,
357,
3103,
18797,
40596,
7,
16,
828,
9529,
82,
986,
4008,
198,
220,
220,
220,
264,
796,
32815,
7,
69,
11,
47764,
11,
2746,
13,
3642,
5685,
82,
8,
198,
220,
220,
220,
277,
796,
4174,
62,
15952,
2611,
7,
69,
11,
264,
11,
10832,
18610,
17633,
11,
468,
62,
5036,
62,
3849,
984,
8,
198,
220,
220,
220,
285,
69,
796,
9104,
19778,
7,
69,
11,
264,
11,
33220,
13,
4033,
388,
429,
540,
7,
7568,
58,
28265,
651,
26745,
12195,
13083,
7,
82,
828,
1058,
37047,
15437,
828,
10832,
18610,
17633,
8,
198,
220,
220,
220,
1303,
33482,
2746,
6759,
8609,
198,
220,
220,
220,
8333,
796,
355,
4593,
7,
69,
8,
198,
220,
220,
220,
468,
62,
5036,
62,
3849,
984,
11405,
1461,
11085,
0,
7,
562,
570,
8,
198,
220,
220,
220,
8085,
796,
9104,
46912,
7,
1952,
7,
43879,
2414,
11,
352,
11,
352,
828,
8333,
8,
198,
220,
220,
220,
8655,
8081,
2234,
17633,
7,
19849,
11,
285,
69,
11,
8085,
8,
198,
437,
198,
198,
2,
38093,
23926,
198,
2,
25048,
649,
4981,
198,
37811,
198,
220,
220,
220,
281,
10071,
62,
1652,
68,
7,
69,
3712,
8479,
4712,
40596,
11,
47764,
11,
410,
66,
709,
3712,
34,
709,
2743,
590,
22362,
320,
1352,
796,
569,
66,
709,
13,
36439,
9783,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1332,
3712,
6030,
90,
27,
25,
4599,
1108,
5189,
31805,
92,
796,
376,
14402,
11,
1279,
2539,
4775,
7159,
43734,
198,
220,
220,
220,
281,
10071,
62,
1652,
68,
7,
9288,
3712,
6030,
90,
27,
25,
4599,
1108,
5189,
31805,
5512,
277,
3712,
8479,
4712,
40596,
11,
47764,
11,
410,
66,
709,
3712,
34,
709,
2743,
590,
22362,
320,
1352,
796,
569,
66,
709,
13,
36439,
9783,
1279,
2539,
4775,
7159,
43734,
198,
198,
1565,
41576,
329,
5969,
12,
10760,
14174,
20683,
13,
198,
9,
4600,
28435,
709,
63,
25,
3959,
1352,
286,
44829,
590,
17593,
13,
198,
9,
4600,
4906,
63,
25,
2099,
286,
281,
10071,
13,
198,
198,
63,
40993,
62,
1652,
68,
63,
7716,
257,
4600,
10962,
8081,
2234,
17633,
90,
27,
25,
10832,
18610,
17633,
92,
44646,
198,
37811,
198,
40993,
62,
1652,
68,
7,
69,
3712,
8479,
4712,
40596,
11,
47764,
11,
410,
66,
709,
3712,
34,
709,
2743,
590,
22362,
320,
1352,
796,
569,
66,
709,
13,
36439,
9783,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1332,
3712,
6030,
90,
27,
25,
4599,
1108,
5189,
31805,
92,
796,
376,
14402,
11,
220,
198,
220,
220,
220,
220,
220,
220,
220,
479,
86,
22046,
23029,
28,
220,
198,
220,
220,
220,
281,
10071,
7,
9288,
11,
10832,
18610,
17633,
11,
277,
11,
47764,
11,
410,
66,
709,
26,
479,
86,
22046,
23029,
198,
198,
40993,
62,
1652,
68,
7,
9288,
3712,
6030,
90,
27,
25,
4599,
1108,
5189,
31805,
5512,
277,
3712,
8479,
4712,
40596,
11,
47764,
11,
410,
66,
709,
3712,
34,
709,
2743,
590,
22362,
320,
1352,
796,
569,
66,
709,
13,
36439,
9783,
479,
86,
22046,
23029,
796,
220,
198,
220,
220,
220,
281,
10071,
7,
9288,
11,
10832,
18610,
17633,
11,
277,
11,
47764,
11,
410,
66,
709,
26,
479,
86,
22046,
23029,
198,
198,
8818,
281,
10071,
7,
9288,
3712,
6030,
90,
27,
25,
4599,
1108,
5189,
31805,
5512,
7904,
6030,
90,
13715,
18610,
17633,
5512,
277,
3712,
8479,
4712,
40596,
11,
47764,
11,
410,
66,
709,
3712,
34,
709,
2743,
590,
22362,
320,
1352,
796,
569,
66,
709,
13,
36439,
9783,
220,
198,
220,
220,
220,
220,
220,
220,
220,
2099,
3712,
5317,
796,
352,
11,
220,
198,
220,
220,
220,
220,
220,
220,
220,
479,
86,
22046,
23029,
198,
220,
220,
220,
491,
76,
796,
284,
62,
2213,
76,
7,
2301,
7,
7568,
11,
277,
11,
410,
66,
709,
26,
479,
86,
22046,
986,
828,
47764,
8,
198,
220,
220,
220,
281,
10071,
7,
9288,
11,
491,
76,
26,
2099,
8,
198,
437,
628,
198
] | 2.387068 | 2,227 |
using Diagonalizations, LinearAlgebra, PosDefManifold, Test
# Method (1) real
t, n, k=10, 20, 10
Xset = [genDataMatrix(t, n) for i = 1:k]
Xfixed=randn(t, n)./1
for i=1:length(Xset) Xset[i]+=Xfixed end
C1=Hermitian( mean((X'*X)/t for X∈Xset) )
C2=Hermitian( mean((X*X')/n for X∈Xset) )
Xbar=mean(Xset)
c=cstp(Xbar, C1, C2; simple=true)
@test c.F[1]'*C2*c.F[1]≈I
@test c.F[2]'*C1*c.F[2]≈I
Z=c.F[1]'*Xbar*c.F[2]
n=minimum(size(Z))
@test norm(Z[1:n, 1:n]-Diagonal(Z[1:n, 1:n]))+1. ≈ 1.
cX=cstp(Xset; simple=true)
@test c==cX
# Method (1) complex
t, n, k=10, 20, 10
Xcset = [genDataMatrix(ComplexF64, t, n) for i = 1:k]
Xcfixed=randn(ComplexF64, t, n)./1
for i=1:length(Xcset) Xcset[i]+=Xcfixed end
C1c=Hermitian( mean((Xc'*Xc)/t for Xc∈Xcset) )
C2c=Hermitian( mean((Xc*Xc')/n for Xc∈Xcset) )
Xcbar=mean(Xcset)
cc=cstp(Xcbar, C1c, C2c; simple=true)
@test cc.F[1]'*C2c*cc.F[1]≈I
@test cc.F[2]'*C1c*cc.F[2]≈I
Zc=cc.F[1]'*Xcbar*cc.F[2]
n=minimum(size(Zc))
@test norm(Zc[1:n, 1:n]-Diagonal(Zc[1:n, 1:n]))+1. ≈ 1.
cXc=cstp(Xcset; simple=true)
@test cc==cXc
# Method (2) real
c=cstp(Xset)
# ... selecting subspace dimension allowing an explained variance = 0.9
c=cstp(Xset; eVar=0.9)
# ... giving weights `w` to the covariance matrices
c=cstp(Xset; w=abs2.(randn(k)), eVar=0.9)
# ... subtracting the means
c=cstp(Xset; meanXd₁=nothing, meanXd₂=nothing, w=abs2.(randn(k)), eVar=0.9)
# explained variance
c.eVar
# name of the filter
c.name
using Plots
# plot the original covariance matrices and the transformed counterpart
c=cstp(Xset)
C1Max=maximum(abs.(C1));
h1 = heatmap(C1, clim=(-C1Max, C1Max), title="C1", yflip=true, c=:bluesreds);
D1=c.F[1]'*C2*c.F[1];
D1Max=maximum(abs.(D1));
h2 = heatmap(D1, clim=(0, D1Max), title="F[1]'*C2*F[1]", yflip=true, c=:amp);
C2Max=maximum(abs.(C2));
h3 = heatmap(C2, clim=(-C2Max, C2Max), title="C2", yflip=true, c=:bluesreds);
D2=c.F[2]'*C1*c.F[2];
D2Max=maximum(abs.(D2));
h4 = heatmap(D2, clim=(0, D2Max), title="F[2]'*C1*F[2]", yflip=true, c=:amp);
XbarMax=maximum(abs.(Xbar));
h5 = heatmap(Xbar, clim=(-XbarMax, XbarMax), title="Xbar", yflip=true, c=:bluesreds);
DX=c.F[1]'*Xbar*c.F[2];
DXMax=maximum(abs.(DX));
h6 = heatmap(DX, clim=(0, DXMax), title="F[1]'*Xbar*F[2]", yflip=true, c=:amp);
📈=plot(h1, h3, h5, h2, h4, h6, size=(800,400))
# savefig(📈, homedir()*"\\Documents\\Code\\julia\\Diagonalizations\\docs\\src\\assets\\FigCSTP.png")
# Method (2) complex
cc=cstp(Xcset)
# ... selecting subspace dimension allowing an explained variance = 0.9
cc=cstp(Xcset; eVar=0.9)
# ... giving weights `w` to the covariance matrices
cc=cstp(Xcset; w=abs2.(randn(k)), eVar=0.9)
# ... subtracting the mean
cc=cstp(Xcset; meanXd₁=nothing, meanXd₂=nothing,
w=abs2.(randn(k)), eVar=0.9)
# explained variance
c.eVar
# name of the filter
c.name
| [
3500,
6031,
27923,
4582,
11,
44800,
2348,
29230,
11,
18574,
7469,
5124,
361,
727,
11,
6208,
198,
198,
2,
11789,
357,
16,
8,
1103,
198,
83,
11,
299,
11,
479,
28,
940,
11,
1160,
11,
838,
198,
55,
2617,
796,
685,
5235,
6601,
46912,
7,
83,
11,
299,
8,
329,
1312,
796,
352,
25,
74,
60,
198,
55,
34021,
28,
25192,
77,
7,
83,
11,
299,
737,
14,
16,
198,
1640,
1312,
28,
16,
25,
13664,
7,
55,
2617,
8,
1395,
2617,
58,
72,
60,
47932,
55,
34021,
886,
198,
34,
16,
28,
9360,
2781,
666,
7,
1612,
19510,
55,
6,
9,
55,
20679,
83,
329,
1395,
24861,
230,
55,
2617,
8,
1267,
198,
34,
17,
28,
9360,
2781,
666,
7,
1612,
19510,
55,
9,
55,
11537,
14,
77,
329,
1395,
24861,
230,
55,
2617,
8,
1267,
198,
55,
5657,
28,
32604,
7,
55,
2617,
8,
198,
66,
28,
66,
301,
79,
7,
55,
5657,
11,
327,
16,
11,
327,
17,
26,
2829,
28,
7942,
8,
198,
31,
9288,
269,
13,
37,
58,
16,
49946,
9,
34,
17,
9,
66,
13,
37,
58,
16,
60,
35705,
230,
40,
198,
31,
9288,
269,
13,
37,
58,
17,
49946,
9,
34,
16,
9,
66,
13,
37,
58,
17,
60,
35705,
230,
40,
198,
57,
28,
66,
13,
37,
58,
16,
49946,
9,
55,
5657,
9,
66,
13,
37,
58,
17,
60,
198,
77,
28,
39504,
7,
7857,
7,
57,
4008,
198,
31,
9288,
2593,
7,
57,
58,
16,
25,
77,
11,
352,
25,
77,
45297,
18683,
27923,
7,
57,
58,
16,
25,
77,
11,
352,
25,
77,
60,
4008,
10,
16,
13,
15139,
230,
352,
13,
198,
66,
55,
28,
66,
301,
79,
7,
55,
2617,
26,
2829,
28,
7942,
8,
198,
31,
9288,
269,
855,
66,
55,
198,
198,
2,
11789,
357,
16,
8,
3716,
198,
83,
11,
299,
11,
479,
28,
940,
11,
1160,
11,
838,
198,
55,
66,
2617,
796,
685,
5235,
6601,
46912,
7,
5377,
11141,
37,
2414,
11,
256,
11,
299,
8,
329,
1312,
796,
352,
25,
74,
60,
198,
55,
12993,
2966,
28,
25192,
77,
7,
5377,
11141,
37,
2414,
11,
256,
11,
299,
737,
14,
16,
198,
1640,
1312,
28,
16,
25,
13664,
7,
55,
66,
2617,
8,
1395,
66,
2617,
58,
72,
60,
47932,
55,
12993,
2966,
886,
198,
34,
16,
66,
28,
9360,
2781,
666,
7,
1612,
19510,
55,
66,
6,
9,
55,
66,
20679,
83,
329,
1395,
66,
24861,
230,
55,
66,
2617,
8,
1267,
198,
34,
17,
66,
28,
9360,
2781,
666,
7,
1612,
19510,
55,
66,
9,
55,
66,
11537,
14,
77,
329,
1395,
66,
24861,
230,
55,
66,
2617,
8,
1267,
198,
55,
66,
5657,
28,
32604,
7,
55,
66,
2617,
8,
198,
535,
28,
66,
301,
79,
7,
55,
66,
5657,
11,
327,
16,
66,
11,
327,
17,
66,
26,
2829,
28,
7942,
8,
198,
31,
9288,
36624,
13,
37,
58,
16,
49946,
9,
34,
17,
66,
9,
535,
13,
37,
58,
16,
60,
35705,
230,
40,
198,
31,
9288,
36624,
13,
37,
58,
17,
49946,
9,
34,
16,
66,
9,
535,
13,
37,
58,
17,
60,
35705,
230,
40,
198,
57,
66,
28,
535,
13,
37,
58,
16,
49946,
9,
55,
66,
5657,
9,
535,
13,
37,
58,
17,
60,
198,
77,
28,
39504,
7,
7857,
7,
57,
66,
4008,
198,
31,
9288,
2593,
7,
57,
66,
58,
16,
25,
77,
11,
352,
25,
77,
45297,
18683,
27923,
7,
57,
66,
58,
16,
25,
77,
11,
352,
25,
77,
60,
4008,
10,
16,
13,
15139,
230,
352,
13,
198,
66,
55,
66,
28,
66,
301,
79,
7,
55,
66,
2617,
26,
2829,
28,
7942,
8,
198,
31,
9288,
36624,
855,
66,
55,
66,
198,
198,
2,
11789,
357,
17,
8,
1103,
198,
66,
28,
66,
301,
79,
7,
55,
2617,
8,
198,
198,
2,
2644,
17246,
850,
13200,
15793,
5086,
281,
4893,
24198,
796,
657,
13,
24,
198,
66,
28,
66,
301,
79,
7,
55,
2617,
26,
304,
19852,
28,
15,
13,
24,
8,
198,
198,
2,
2644,
3501,
19590,
4600,
86,
63,
284,
262,
44829,
590,
2603,
45977,
198,
66,
28,
66,
301,
79,
7,
55,
2617,
26,
266,
28,
8937,
17,
12195,
25192,
77,
7,
74,
36911,
304,
19852,
28,
15,
13,
24,
8,
198,
198,
2,
2644,
34128,
278,
262,
1724,
198,
66,
28,
66,
301,
79,
7,
55,
2617,
26,
1612,
55,
67,
158,
224,
223,
28,
22366,
11,
1612,
55,
67,
158,
224,
224,
28,
22366,
11,
266,
28,
8937,
17,
12195,
25192,
77,
7,
74,
36911,
304,
19852,
28,
15,
13,
24,
8,
198,
198,
2,
4893,
24198,
198,
66,
13,
68,
19852,
198,
198,
2,
1438,
286,
262,
8106,
198,
66,
13,
3672,
198,
198,
3500,
1345,
1747,
198,
2,
7110,
262,
2656,
44829,
590,
2603,
45977,
290,
262,
14434,
11283,
198,
66,
28,
66,
301,
79,
7,
55,
2617,
8,
198,
198,
34,
16,
11518,
28,
47033,
7,
8937,
12195,
34,
16,
18125,
198,
289,
16,
796,
4894,
8899,
7,
34,
16,
11,
5424,
16193,
12,
34,
16,
11518,
11,
327,
16,
11518,
828,
3670,
2625,
34,
16,
1600,
331,
2704,
541,
28,
7942,
11,
269,
28,
25,
2436,
947,
445,
82,
1776,
198,
360,
16,
28,
66,
13,
37,
58,
16,
49946,
9,
34,
17,
9,
66,
13,
37,
58,
16,
11208,
198,
360,
16,
11518,
28,
47033,
7,
8937,
12195,
35,
16,
18125,
198,
289,
17,
796,
4894,
8899,
7,
35,
16,
11,
5424,
16193,
15,
11,
360,
16,
11518,
828,
3670,
2625,
37,
58,
16,
49946,
9,
34,
17,
9,
37,
58,
16,
60,
1600,
331,
2704,
541,
28,
7942,
11,
269,
28,
25,
696,
1776,
198,
327,
17,
11518,
28,
47033,
7,
8937,
12195,
34,
17,
18125,
198,
289,
18,
796,
4894,
8899,
7,
34,
17,
11,
5424,
16193,
12,
34,
17,
11518,
11,
327,
17,
11518,
828,
3670,
2625,
34,
17,
1600,
331,
2704,
541,
28,
7942,
11,
269,
28,
25,
2436,
947,
445,
82,
1776,
198,
360,
17,
28,
66,
13,
37,
58,
17,
49946,
9,
34,
16,
9,
66,
13,
37,
58,
17,
11208,
198,
360,
17,
11518,
28,
47033,
7,
8937,
12195,
35,
17,
18125,
198,
289,
19,
796,
4894,
8899,
7,
35,
17,
11,
5424,
16193,
15,
11,
360,
17,
11518,
828,
3670,
2625,
37,
58,
17,
49946,
9,
34,
16,
9,
37,
58,
17,
60,
1600,
331,
2704,
541,
28,
7942,
11,
269,
28,
25,
696,
1776,
198,
198,
55,
5657,
11518,
28,
47033,
7,
8937,
12195,
55,
5657,
18125,
198,
289,
20,
796,
4894,
8899,
7,
55,
5657,
11,
5424,
16193,
12,
55,
5657,
11518,
11,
1395,
5657,
11518,
828,
3670,
2625,
55,
5657,
1600,
331,
2704,
541,
28,
7942,
11,
269,
28,
25,
2436,
947,
445,
82,
1776,
198,
19393,
28,
66,
13,
37,
58,
16,
49946,
9,
55,
5657,
9,
66,
13,
37,
58,
17,
11208,
198,
19393,
11518,
28,
47033,
7,
8937,
12195,
36227,
18125,
198,
289,
21,
796,
4894,
8899,
7,
36227,
11,
5424,
16193,
15,
11,
19393,
11518,
828,
3670,
2625,
37,
58,
16,
49946,
9,
55,
5657,
9,
37,
58,
17,
60,
1600,
331,
2704,
541,
28,
7942,
11,
269,
28,
25,
696,
1776,
198,
12520,
241,
230,
28,
29487,
7,
71,
16,
11,
289,
18,
11,
289,
20,
11,
289,
17,
11,
289,
19,
11,
289,
21,
11,
2546,
16193,
7410,
11,
7029,
4008,
198,
2,
3613,
5647,
7,
8582,
241,
230,
11,
3488,
276,
343,
3419,
9,
1,
6852,
38354,
6852,
10669,
6852,
73,
43640,
6852,
18683,
27923,
4582,
6852,
31628,
6852,
10677,
6852,
19668,
6852,
14989,
34,
2257,
47,
13,
11134,
4943,
198,
198,
2,
11789,
357,
17,
8,
3716,
198,
535,
28,
66,
301,
79,
7,
55,
66,
2617,
8,
198,
198,
2,
2644,
17246,
850,
13200,
15793,
5086,
281,
4893,
24198,
796,
657,
13,
24,
198,
535,
28,
66,
301,
79,
7,
55,
66,
2617,
26,
304,
19852,
28,
15,
13,
24,
8,
198,
198,
2,
2644,
3501,
19590,
4600,
86,
63,
284,
262,
44829,
590,
2603,
45977,
198,
535,
28,
66,
301,
79,
7,
55,
66,
2617,
26,
266,
28,
8937,
17,
12195,
25192,
77,
7,
74,
36911,
304,
19852,
28,
15,
13,
24,
8,
198,
198,
2,
2644,
34128,
278,
262,
1612,
198,
535,
28,
66,
301,
79,
7,
55,
66,
2617,
26,
1612,
55,
67,
158,
224,
223,
28,
22366,
11,
1612,
55,
67,
158,
224,
224,
28,
22366,
11,
198,
220,
220,
220,
220,
220,
220,
220,
266,
28,
8937,
17,
12195,
25192,
77,
7,
74,
36911,
304,
19852,
28,
15,
13,
24,
8,
198,
198,
2,
4893,
24198,
198,
66,
13,
68,
19852,
198,
198,
2,
1438,
286,
262,
8106,
198,
66,
13,
3672,
198
] | 1.941095 | 1,443 |
using SparseVectors
using Base.Test
import SparseVectors: exact_equal
### Data
rnd_x0 = sprand(50, 0.6)
rnd_x0f = full(rnd_x0)
rnd_x1 = sprand(50, 0.7) * 4.0
rnd_x1f = full(rnd_x1)
spv_x1 = SparseVector(8, [2, 5, 6], [1.25, -0.75, 3.5])
_x2 = SparseVector(8, [1, 2, 6, 7], [3.25, 4.0, -5.5, -6.0])
spv_x2 = view(_x2)
### Arithmetic operations
let x = spv_x1, x2 = spv_x2
# negate
@test exact_equal(-x, SparseVector(8, [2, 5, 6], [-1.25, 0.75, -3.5]))
# abs and abs2
@test exact_equal(abs(x), SparseVector(8, [2, 5, 6], abs([1.25, -0.75, 3.5])))
@test exact_equal(abs2(x), SparseVector(8, [2, 5, 6], abs2([1.25, -0.75, 3.5])))
# plus and minus
xa = SparseVector(8, [1,2,5,6,7], [3.25,5.25,-0.75,-2.0,-6.0])
@test exact_equal(x + x, x * 2)
@test exact_equal(x + x2, xa)
@test exact_equal(x2 + x, xa)
xb = SparseVector(8, [1,2,5,6,7], [-3.25,-2.75,-0.75,9.0,6.0])
@test exact_equal(x - x, SparseVector(8, Int[], Float64[]))
@test exact_equal(x - x2, xb)
@test exact_equal(x2 - x, -xb)
@test full(x) + x2 == full(xa)
@test full(x) - x2 == full(xb)
@test x + full(x2) == full(xa)
@test x - full(x2) == full(xb)
# multiplies
xm = SparseVector(8, [2, 6], [5.0, -19.25])
@test exact_equal(x .* x, abs2(x))
@test exact_equal(x .* x2, xm)
@test exact_equal(x2 .* x, xm)
@test full(x) .* x2 == full(xm)
@test x .* full(x2) == full(xm)
# max & min
@test exact_equal(max(x, x), x)
@test exact_equal(min(x, x), x)
@test exact_equal(max(x, x2),
SparseVector(8, Int[1, 2, 6], Float64[3.25, 4.0, 3.5]))
@test exact_equal(min(x, x2),
SparseVector(8, Int[2, 5, 6, 7], Float64[1.25, -0.75, -5.5, -6.0]))
end
### Complex
let x = spv_x1, x2 = spv_x2
# complex
@test exact_equal(complex(x, x),
SparseVector(8, [2,5,6], [1.25+1.25im, -0.75-0.75im, 3.5+3.5im]))
@test exact_equal(complex(x, x2),
SparseVector(8, [1,2,5,6,7], [3.25im, 1.25+4.0im, -0.75+0.im, 3.5-5.5im, -6.0im]))
@test exact_equal(complex(x2, x),
SparseVector(8, [1,2,5,6,7], [3.25+0.im, 4.0+1.25im, -0.75im, -5.5+3.5im, -6.0+0.im]))
# real & imag
@test is(real(x), x)
@test exact_equal(imag(x), sparsevector(Float64, length(x)))
xcp = complex(x, x2)
@test exact_equal(real(xcp), x)
@test exact_equal(imag(xcp), x2)
end
### Zero-preserving math functions: sparse -> sparse
function check_nz2z_z2z{T}(f::Function, x::SparseVector{T}, xf::Vector{T})
R = typeof(f(zero(T)))
r = f(x)
isa(r, AbstractSparseVector) || error("$f(x) is not a sparse vector.")
eltype(r) == R || error("$f(x) results in eltype = $(eltype(r)), expect $R")
all(r.nzval .!= 0) || error("$f(x) contains zeros in nzval.")
full(r) == f(xf) || error("Incorrect results found in $f(x).")
end
for f in [floor, ceil, trunc, round]
check_nz2z_z2z(f, rnd_x1, rnd_x1f)
end
for f in [log1p, expm1,
sin, tan, sinpi, sind, tand,
asin, atan, asind, atand,
sinh, tanh, asinh, atanh]
check_nz2z_z2z(f, rnd_x0, rnd_x0f)
end
### Non-zero-preserving math functions: sparse -> dense
function check_z2nz{T}(f::Function, x::SparseVector{T}, xf::Vector{T})
R = typeof(f(zero(T)))
r = f(x)
isa(r, Vector) || error("$f(x) is not a dense vector.")
eltype(r) == R || error("$f(x) results in eltype = $(eltype(r)), expect $R")
r == f(xf) || error("Incorrect results found in $f(x).")
end
for f in [exp, exp2, exp10, log, log2, log10,
cos, csc, cot, sec, cospi,
cosd, cscd, cotd, secd,
acos, acot, acosd, acotd,
cosh, csch, coth, sech, acsch, asech]
check_z2nz(f, rnd_x0, rnd_x0f)
end
### Reduction
# sum, sumabs, sumabs2, vecnorm
let x = spv_x1
@test sum(x) == 4.0
@test sumabs(x) == 5.5
@test sumabs2(x) == 14.375
@test vecnorm(x) == sqrt(14.375)
@test vecnorm(x, 1) == 5.5
@test vecnorm(x, 2) == sqrt(14.375)
@test vecnorm(x, Inf) == 3.5
end
# maximum, minimum, maxabs, minabs
let x = spv_x1
@test maximum(x) == 3.5
@test minimum(x) == -0.75
@test maxabs(x) == 3.5
@test minabs(x) == 0.0
end
let x = abs(spv_x1)
@test maximum(x) == 3.5
@test minimum(x) == 0.0
end
let x = -abs(spv_x1)
@test maximum(x) == 0.0
@test minimum(x) == -3.5
end
let x = SparseVector(3, [1, 2, 3], [-4.5, 2.5, 3.5])
@test maximum(x) == 3.5
@test minimum(x) == -4.5
@test maxabs(x) == 4.5
@test minabs(x) == 2.5
end
let x = sparsevector(Float64, 8)
@test maximum(x) == 0.0
@test minimum(x) == 0.0
@test maxabs(x) == 0.0
@test minabs(x) == 0.0
end
# Issue https://github.com/JuliaLang/julia/issues/14046
let s14046 = sprand(5, 1.0)
z = sparsevector(Float64, 5)
@test z + s14046 == s14046
@test 2*z == z + z == z
end
| [
3500,
1338,
17208,
53,
478,
669,
198,
3500,
7308,
13,
14402,
198,
198,
11748,
1338,
17208,
53,
478,
669,
25,
2748,
62,
40496,
198,
198,
21017,
6060,
198,
198,
81,
358,
62,
87,
15,
796,
7500,
392,
7,
1120,
11,
657,
13,
21,
8,
198,
81,
358,
62,
87,
15,
69,
796,
1336,
7,
81,
358,
62,
87,
15,
8,
198,
198,
81,
358,
62,
87,
16,
796,
7500,
392,
7,
1120,
11,
657,
13,
22,
8,
1635,
604,
13,
15,
198,
81,
358,
62,
87,
16,
69,
796,
1336,
7,
81,
358,
62,
87,
16,
8,
198,
198,
2777,
85,
62,
87,
16,
796,
1338,
17208,
38469,
7,
23,
11,
685,
17,
11,
642,
11,
718,
4357,
685,
16,
13,
1495,
11,
532,
15,
13,
2425,
11,
513,
13,
20,
12962,
198,
62,
87,
17,
796,
1338,
17208,
38469,
7,
23,
11,
685,
16,
11,
362,
11,
718,
11,
767,
4357,
685,
18,
13,
1495,
11,
604,
13,
15,
11,
532,
20,
13,
20,
11,
532,
21,
13,
15,
12962,
198,
2777,
85,
62,
87,
17,
796,
1570,
28264,
87,
17,
8,
628,
198,
21017,
943,
29848,
4560,
198,
198,
1616,
2124,
796,
599,
85,
62,
87,
16,
11,
2124,
17,
796,
599,
85,
62,
87,
17,
198,
220,
220,
220,
1303,
44328,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
32590,
87,
11,
1338,
17208,
38469,
7,
23,
11,
685,
17,
11,
642,
11,
718,
4357,
25915,
16,
13,
1495,
11,
657,
13,
2425,
11,
532,
18,
13,
20,
60,
4008,
628,
220,
220,
220,
1303,
2352,
290,
2352,
17,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
8937,
7,
87,
828,
1338,
17208,
38469,
7,
23,
11,
685,
17,
11,
642,
11,
718,
4357,
2352,
26933,
16,
13,
1495,
11,
532,
15,
13,
2425,
11,
513,
13,
20,
60,
22305,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
8937,
17,
7,
87,
828,
1338,
17208,
38469,
7,
23,
11,
685,
17,
11,
642,
11,
718,
4357,
2352,
17,
26933,
16,
13,
1495,
11,
532,
15,
13,
2425,
11,
513,
13,
20,
60,
22305,
628,
220,
220,
220,
1303,
5556,
290,
20208,
198,
220,
220,
220,
2124,
64,
796,
1338,
17208,
38469,
7,
23,
11,
685,
16,
11,
17,
11,
20,
11,
21,
11,
22,
4357,
685,
18,
13,
1495,
11,
20,
13,
1495,
12095,
15,
13,
2425,
12095,
17,
13,
15,
12095,
21,
13,
15,
12962,
628,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
87,
1343,
2124,
11,
2124,
1635,
362,
8,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
87,
1343,
2124,
17,
11,
2124,
64,
8,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
87,
17,
1343,
2124,
11,
2124,
64,
8,
628,
220,
220,
220,
2124,
65,
796,
1338,
17208,
38469,
7,
23,
11,
685,
16,
11,
17,
11,
20,
11,
21,
11,
22,
4357,
25915,
18,
13,
1495,
12095,
17,
13,
2425,
12095,
15,
13,
2425,
11,
24,
13,
15,
11,
21,
13,
15,
12962,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
87,
532,
2124,
11,
1338,
17208,
38469,
7,
23,
11,
2558,
58,
4357,
48436,
2414,
21737,
4008,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
87,
532,
2124,
17,
11,
2124,
65,
8,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
87,
17,
532,
2124,
11,
532,
30894,
8,
628,
220,
220,
220,
2488,
9288,
1336,
7,
87,
8,
1343,
2124,
17,
6624,
1336,
7,
27865,
8,
198,
220,
220,
220,
2488,
9288,
1336,
7,
87,
8,
532,
2124,
17,
6624,
1336,
7,
30894,
8,
198,
220,
220,
220,
2488,
9288,
2124,
1343,
1336,
7,
87,
17,
8,
6624,
1336,
7,
27865,
8,
198,
220,
220,
220,
2488,
9288,
2124,
532,
1336,
7,
87,
17,
8,
6624,
1336,
7,
30894,
8,
628,
220,
220,
220,
1303,
15082,
444,
198,
220,
220,
220,
2124,
76,
796,
1338,
17208,
38469,
7,
23,
11,
685,
17,
11,
718,
4357,
685,
20,
13,
15,
11,
532,
1129,
13,
1495,
12962,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
87,
764,
9,
2124,
11,
2352,
17,
7,
87,
4008,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
87,
764,
9,
2124,
17,
11,
2124,
76,
8,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
87,
17,
764,
9,
2124,
11,
2124,
76,
8,
628,
220,
220,
220,
2488,
9288,
1336,
7,
87,
8,
764,
9,
2124,
17,
6624,
1336,
7,
87,
76,
8,
198,
220,
220,
220,
2488,
9288,
2124,
764,
9,
1336,
7,
87,
17,
8,
6624,
1336,
7,
87,
76,
8,
628,
220,
220,
220,
1303,
3509,
1222,
949,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
9806,
7,
87,
11,
2124,
828,
2124,
8,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
1084,
7,
87,
11,
2124,
828,
2124,
8,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
9806,
7,
87,
11,
2124,
17,
828,
198,
220,
220,
220,
220,
220,
220,
220,
1338,
17208,
38469,
7,
23,
11,
2558,
58,
16,
11,
362,
11,
718,
4357,
48436,
2414,
58,
18,
13,
1495,
11,
604,
13,
15,
11,
513,
13,
20,
60,
4008,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
1084,
7,
87,
11,
2124,
17,
828,
198,
220,
220,
220,
220,
220,
220,
220,
1338,
17208,
38469,
7,
23,
11,
2558,
58,
17,
11,
642,
11,
718,
11,
767,
4357,
48436,
2414,
58,
16,
13,
1495,
11,
532,
15,
13,
2425,
11,
532,
20,
13,
20,
11,
532,
21,
13,
15,
60,
4008,
198,
437,
198,
198,
21017,
19157,
198,
198,
1616,
2124,
796,
599,
85,
62,
87,
16,
11,
2124,
17,
796,
599,
85,
62,
87,
17,
198,
220,
220,
220,
1303,
3716,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
41887,
7,
87,
11,
2124,
828,
198,
220,
220,
220,
220,
220,
220,
220,
1338,
17208,
38469,
7,
23,
11,
685,
17,
11,
20,
11,
21,
4357,
685,
16,
13,
1495,
10,
16,
13,
1495,
320,
11,
532,
15,
13,
2425,
12,
15,
13,
2425,
320,
11,
513,
13,
20,
10,
18,
13,
20,
320,
60,
4008,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
41887,
7,
87,
11,
2124,
17,
828,
198,
220,
220,
220,
220,
220,
220,
220,
1338,
17208,
38469,
7,
23,
11,
685,
16,
11,
17,
11,
20,
11,
21,
11,
22,
4357,
685,
18,
13,
1495,
320,
11,
352,
13,
1495,
10,
19,
13,
15,
320,
11,
532,
15,
13,
2425,
10,
15,
13,
320,
11,
513,
13,
20,
12,
20,
13,
20,
320,
11,
532,
21,
13,
15,
320,
60,
4008,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
41887,
7,
87,
17,
11,
2124,
828,
198,
220,
220,
220,
220,
220,
220,
220,
1338,
17208,
38469,
7,
23,
11,
685,
16,
11,
17,
11,
20,
11,
21,
11,
22,
4357,
685,
18,
13,
1495,
10,
15,
13,
320,
11,
604,
13,
15,
10,
16,
13,
1495,
320,
11,
532,
15,
13,
2425,
320,
11,
532,
20,
13,
20,
10,
18,
13,
20,
320,
11,
532,
21,
13,
15,
10,
15,
13,
320,
60,
4008,
628,
220,
220,
220,
1303,
1103,
1222,
3590,
628,
220,
220,
220,
2488,
9288,
318,
7,
5305,
7,
87,
828,
2124,
8,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
48466,
7,
87,
828,
29877,
31364,
7,
43879,
2414,
11,
4129,
7,
87,
22305,
628,
220,
220,
220,
2124,
13155,
796,
3716,
7,
87,
11,
2124,
17,
8,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
5305,
7,
87,
13155,
828,
2124,
8,
198,
220,
220,
220,
2488,
9288,
2748,
62,
40496,
7,
48466,
7,
87,
13155,
828,
2124,
17,
8,
198,
437,
198,
198,
21017,
12169,
12,
18302,
14344,
10688,
5499,
25,
29877,
4613,
29877,
198,
198,
8818,
2198,
62,
27305,
17,
89,
62,
89,
17,
89,
90,
51,
92,
7,
69,
3712,
22203,
11,
2124,
3712,
50,
29572,
38469,
90,
51,
5512,
2124,
69,
3712,
38469,
90,
51,
30072,
198,
220,
220,
220,
371,
796,
2099,
1659,
7,
69,
7,
22570,
7,
51,
22305,
198,
220,
220,
220,
374,
796,
277,
7,
87,
8,
198,
220,
220,
220,
318,
64,
7,
81,
11,
27741,
50,
29572,
38469,
8,
8614,
4049,
7203,
3,
69,
7,
87,
8,
318,
407,
257,
29877,
15879,
19570,
198,
220,
220,
220,
1288,
4906,
7,
81,
8,
6624,
371,
8614,
4049,
7203,
3,
69,
7,
87,
8,
2482,
287,
1288,
4906,
796,
29568,
417,
4906,
7,
81,
36911,
1607,
720,
49,
4943,
198,
220,
220,
220,
477,
7,
81,
13,
27305,
2100,
764,
0,
28,
657,
8,
8614,
4049,
7203,
3,
69,
7,
87,
8,
4909,
1976,
27498,
287,
299,
89,
2100,
19570,
198,
220,
220,
220,
1336,
7,
81,
8,
6624,
277,
7,
26152,
8,
8614,
4049,
7203,
818,
30283,
2482,
1043,
287,
720,
69,
7,
87,
8,
19570,
198,
437,
198,
198,
1640,
277,
287,
685,
28300,
11,
2906,
346,
11,
40122,
11,
2835,
60,
198,
220,
220,
220,
2198,
62,
27305,
17,
89,
62,
89,
17,
89,
7,
69,
11,
374,
358,
62,
87,
16,
11,
374,
358,
62,
87,
16,
69,
8,
198,
437,
198,
198,
1640,
277,
287,
685,
6404,
16,
79,
11,
1033,
76,
16,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7813,
11,
25706,
11,
7813,
14415,
11,
264,
521,
11,
256,
392,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
355,
259,
11,
379,
272,
11,
355,
521,
11,
379,
392,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7813,
71,
11,
25706,
71,
11,
355,
259,
71,
11,
379,
272,
71,
60,
198,
220,
220,
220,
2198,
62,
27305,
17,
89,
62,
89,
17,
89,
7,
69,
11,
374,
358,
62,
87,
15,
11,
374,
358,
62,
87,
15,
69,
8,
198,
437,
198,
198,
21017,
8504,
12,
22570,
12,
18302,
14344,
10688,
5499,
25,
29877,
4613,
15715,
198,
198,
8818,
2198,
62,
89,
17,
27305,
90,
51,
92,
7,
69,
3712,
22203,
11,
2124,
3712,
50,
29572,
38469,
90,
51,
5512,
2124,
69,
3712,
38469,
90,
51,
30072,
198,
220,
220,
220,
371,
796,
2099,
1659,
7,
69,
7,
22570,
7,
51,
22305,
198,
220,
220,
220,
374,
796,
277,
7,
87,
8,
198,
220,
220,
220,
318,
64,
7,
81,
11,
20650,
8,
8614,
4049,
7203,
3,
69,
7,
87,
8,
318,
407,
257,
15715,
15879,
19570,
198,
220,
220,
220,
1288,
4906,
7,
81,
8,
6624,
371,
8614,
4049,
7203,
3,
69,
7,
87,
8,
2482,
287,
1288,
4906,
796,
29568,
417,
4906,
7,
81,
36911,
1607,
720,
49,
4943,
198,
220,
220,
220,
374,
6624,
277,
7,
26152,
8,
8614,
4049,
7203,
818,
30283,
2482,
1043,
287,
720,
69,
7,
87,
8,
19570,
198,
437,
198,
198,
1640,
277,
287,
685,
11201,
11,
1033,
17,
11,
1033,
940,
11,
2604,
11,
2604,
17,
11,
2604,
940,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
8615,
11,
269,
1416,
11,
269,
313,
11,
792,
11,
269,
2117,
72,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
8615,
67,
11,
269,
1416,
67,
11,
269,
313,
67,
11,
792,
67,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
936,
418,
11,
936,
313,
11,
936,
418,
67,
11,
936,
313,
67,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
3768,
11,
269,
20601,
11,
269,
849,
11,
384,
354,
11,
936,
20601,
11,
257,
325,
354,
60,
198,
220,
220,
220,
2198,
62,
89,
17,
27305,
7,
69,
11,
374,
358,
62,
87,
15,
11,
374,
358,
62,
87,
15,
69,
8,
198,
437,
628,
198,
21017,
33396,
198,
198,
2,
2160,
11,
2160,
8937,
11,
2160,
8937,
17,
11,
43030,
27237,
198,
198,
1616,
2124,
796,
599,
85,
62,
87,
16,
198,
220,
220,
220,
2488,
9288,
2160,
7,
87,
8,
6624,
604,
13,
15,
198,
220,
220,
220,
2488,
9288,
2160,
8937,
7,
87,
8,
6624,
642,
13,
20,
198,
220,
220,
220,
2488,
9288,
2160,
8937,
17,
7,
87,
8,
6624,
1478,
13,
22318,
628,
220,
220,
220,
2488,
9288,
43030,
27237,
7,
87,
8,
6624,
19862,
17034,
7,
1415,
13,
22318,
8,
198,
220,
220,
220,
2488,
9288,
43030,
27237,
7,
87,
11,
352,
8,
6624,
642,
13,
20,
198,
220,
220,
220,
2488,
9288,
43030,
27237,
7,
87,
11,
362,
8,
6624,
19862,
17034,
7,
1415,
13,
22318,
8,
198,
220,
220,
220,
2488,
9288,
43030,
27237,
7,
87,
11,
4806,
8,
6624,
513,
13,
20,
198,
437,
198,
198,
2,
5415,
11,
5288,
11,
3509,
8937,
11,
949,
8937,
198,
198,
1616,
2124,
796,
599,
85,
62,
87,
16,
198,
220,
220,
220,
2488,
9288,
5415,
7,
87,
8,
6624,
513,
13,
20,
198,
220,
220,
220,
2488,
9288,
5288,
7,
87,
8,
6624,
532,
15,
13,
2425,
198,
220,
220,
220,
2488,
9288,
3509,
8937,
7,
87,
8,
6624,
513,
13,
20,
198,
220,
220,
220,
2488,
9288,
949,
8937,
7,
87,
8,
6624,
657,
13,
15,
198,
437,
198,
198,
1616,
2124,
796,
2352,
7,
2777,
85,
62,
87,
16,
8,
198,
220,
220,
220,
2488,
9288,
5415,
7,
87,
8,
6624,
513,
13,
20,
198,
220,
220,
220,
2488,
9288,
5288,
7,
87,
8,
6624,
657,
13,
15,
198,
437,
198,
198,
1616,
2124,
796,
532,
8937,
7,
2777,
85,
62,
87,
16,
8,
198,
220,
220,
220,
2488,
9288,
5415,
7,
87,
8,
6624,
657,
13,
15,
198,
220,
220,
220,
2488,
9288,
5288,
7,
87,
8,
6624,
532,
18,
13,
20,
198,
437,
198,
198,
1616,
2124,
796,
1338,
17208,
38469,
7,
18,
11,
685,
16,
11,
362,
11,
513,
4357,
25915,
19,
13,
20,
11,
362,
13,
20,
11,
513,
13,
20,
12962,
198,
220,
220,
220,
2488,
9288,
5415,
7,
87,
8,
6624,
513,
13,
20,
198,
220,
220,
220,
2488,
9288,
5288,
7,
87,
8,
6624,
532,
19,
13,
20,
198,
220,
220,
220,
2488,
9288,
3509,
8937,
7,
87,
8,
6624,
604,
13,
20,
198,
220,
220,
220,
2488,
9288,
949,
8937,
7,
87,
8,
6624,
362,
13,
20,
198,
437,
198,
198,
1616,
2124,
796,
29877,
31364,
7,
43879,
2414,
11,
807,
8,
198,
220,
220,
220,
2488,
9288,
5415,
7,
87,
8,
6624,
657,
13,
15,
198,
220,
220,
220,
2488,
9288,
5288,
7,
87,
8,
6624,
657,
13,
15,
198,
220,
220,
220,
2488,
9288,
3509,
8937,
7,
87,
8,
6624,
657,
13,
15,
198,
220,
220,
220,
2488,
9288,
949,
8937,
7,
87,
8,
6624,
657,
13,
15,
198,
437,
198,
198,
2,
18232,
3740,
1378,
12567,
13,
785,
14,
16980,
544,
43,
648,
14,
73,
43640,
14,
37165,
14,
1415,
45438,
198,
1616,
264,
1415,
45438,
796,
7500,
392,
7,
20,
11,
352,
13,
15,
8,
198,
220,
220,
220,
1976,
796,
29877,
31364,
7,
43879,
2414,
11,
642,
8,
198,
220,
220,
220,
2488,
9288,
1976,
1343,
264,
1415,
45438,
6624,
264,
1415,
45438,
198,
220,
220,
220,
2488,
9288,
362,
9,
89,
6624,
1976,
1343,
1976,
6624,
1976,
198,
437,
198
] | 1.916898 | 2,527 |
import accrue.cryptoerase.runtime.Condition;
class C {
int{L} x = 7;
final int z = m();
static final Condition c = new Condition();
int m() {
int y = ({L /c T}) 42;
return y;
}
public static void main(String[] args) {
C obj = new C();
obj.c.set();
}
}
| [
11748,
697,
24508,
13,
29609,
78,
263,
589,
13,
43282,
13,
48362,
26,
198,
198,
4871,
327,
1391,
198,
220,
220,
220,
493,
90,
43,
92,
2124,
796,
767,
26,
198,
220,
220,
220,
2457,
493,
1976,
796,
285,
9783,
198,
220,
220,
220,
9037,
2457,
24295,
269,
796,
649,
24295,
9783,
198,
220,
220,
220,
220,
198,
220,
220,
220,
493,
285,
3419,
1391,
198,
197,
600,
331,
796,
37913,
43,
1220,
66,
309,
30072,
5433,
26,
198,
197,
7783,
331,
26,
198,
220,
220,
220,
1782,
628,
220,
220,
220,
1171,
9037,
7951,
1388,
7,
10100,
21737,
26498,
8,
1391,
198,
197,
34,
26181,
796,
649,
327,
9783,
198,
197,
26801,
13,
66,
13,
2617,
9783,
198,
220,
220,
220,
1782,
198,
92,
198
] | 2.309524 | 126 |
using Static, Aqua
using Test
@testset "Static.jl" begin
Aqua.test_all(Static)
@testset "StaticInt" begin
@test static(UInt(8)) === StaticInt(UInt(8)) === StaticInt{8}()
@test iszero(StaticInt(0))
@test !iszero(StaticInt(1))
@test !isone(StaticInt(0))
@test isone(StaticInt(1))
@test @inferred(one(StaticInt(1))) === StaticInt(1)
@test @inferred(zero(StaticInt(1))) === StaticInt(0)
@test @inferred(one(StaticInt)) === StaticInt(1)
@test @inferred(zero(StaticInt)) === StaticInt(0) === StaticInt(StaticInt(Val(0)))
@test eltype(one(StaticInt)) <: Int
x = StaticInt(1)
@test @inferred(Bool(x)) isa Bool
@test @inferred(BigInt(x)) isa BigInt
@test @inferred(Integer(x)) === x
@test @inferred(%(x, Integer)) === 1
# test for ambiguities and correctness
for i ∈ Any[StaticInt(0), StaticInt(1), StaticInt(2), 3]
for j ∈ Any[StaticInt(0), StaticInt(1), StaticInt(2), 3]
i === j === 3 && continue
for f ∈ [+, -, *, ÷, %, <<, >>, >>>, &, |, ⊻, ==, ≤, ≥]
(iszero(j) && ((f === ÷) || (f === %))) && continue # integer division error
@test convert(Int, @inferred(f(i,j))) == f(convert(Int, i), convert(Int, j))
end
end
i == 3 && break
for f ∈ [+, -, *, /, ÷, %, ==, ≤, ≥]
w = f(convert(Int, i), 1.4)
x = f(1.4, convert(Int, i))
@test convert(typeof(w), @inferred(f(i, 1.4))) === w
@test convert(typeof(x), @inferred(f(1.4, i))) === x # if f is division and i === StaticInt(0), returns `NaN`; hence use of ==== in check.
(((f === ÷) || (f === %)) && (i === StaticInt(0))) && continue
y = f(convert(Int, i), 2 // 7)
z = f(2 // 7, convert(Int, i))
@test convert(typeof(y), @inferred(f(i, 2 // 7))) === y
@test convert(typeof(z), @inferred(f(2 // 7, i))) === z
end
end
@test UnitRange{Int16}(StaticInt(-9), 17) === Int16(-9):Int16(17)
@test UnitRange{Int16}(-7, StaticInt(19)) === Int16(-7):Int16(19)
@test UnitRange(-11, StaticInt(15)) === -11:15
@test UnitRange(StaticInt(-11), 15) === -11:15
@test UnitRange{Int}(StaticInt(-11), StaticInt(15)) === -11:15
@test UnitRange(StaticInt(-11), StaticInt(15)) === -11:15
@test float(StaticInt(8)) === static(8.0)
# test specific promote rules to ensure we don't cause ambiguities
SI = StaticInt{1}
IR = typeof(1//1)
PI = typeof(pi)
@test @inferred(convert(SI, SI())) === SI()
@test @inferred(promote_rule(SI, PI)) <: promote_type(Int, PI)
@test @inferred(promote_rule(SI, IR)) <: promote_type(Int, IR)
@test @inferred(promote_rule(SI, SI)) <: Int
@test @inferred(promote_rule(Missing, SI)) <: promote_type(Missing, Int)
@test @inferred(promote_rule(Nothing, SI)) <: promote_type(Nothing, Int)
@test @inferred(promote_rule(SI, Missing)) <: promote_type(Int, Missing)
@test @inferred(promote_rule(SI, Nothing)) <: promote_type(Int, Nothing)
@test @inferred(promote_rule(Union{Missing,Int}, SI)) <: promote_type(Union{Missing,Int}, Int)
@test @inferred(promote_rule(Union{Nothing,Int}, SI)) <: promote_type(Union{Nothing,Int}, Int)
@test @inferred(promote_rule(Union{Nothing,Missing,Int}, SI)) <: Union{Nothing,Missing,Int}
@test @inferred(promote_rule(Union{Nothing,Missing}, SI)) <: promote_type(Union{Nothing,Missing}, Int)
@test @inferred(promote_rule(SI, Missing)) <: promote_type(Int, Missing)
@test @inferred(promote_rule(Base.TwicePrecision{Int}, StaticInt{1})) <: Base.TwicePrecision{Int}
@test static(Int8(-18)) === static(-18)
@test static(0xef) === static(239)
@test static(Int16(-18)) === static(-18)
@test static(0xffef) === static(65519)
if sizeof(Int) == 8
@test static(Int32(-18)) === static(-18)
@test static(0xffffffef) === static(4294967279)
end
end
@testset "StaticBool" begin
t = static(static(true))
f = StaticBool(static(false))
@test StaticBool{true}() === t
@test StaticBool{false}() === f
@test @inferred(StaticInt(t)) === StaticInt(1)
@test @inferred(StaticInt(f)) === StaticInt(0)
@test @inferred(~t) === f
@test @inferred(~f) === t
@test @inferred(!t) === f
@test @inferred(!f) === t
@test @inferred(+t) === StaticInt(1)
@test @inferred(+f) === StaticInt(0)
@test @inferred(-t) === StaticInt(-1)
@test @inferred(-f) === StaticInt(0)
@test @inferred(sign(t)) === t
@test @inferred(abs(t)) === t
@test @inferred(abs2(t)) === t
@test @inferred(iszero(t)) === f
@test @inferred(isone(t)) === t
@test @inferred(iszero(f)) === t
@test @inferred(isone(f)) === f
@test @inferred(xor(true, f))
@test @inferred(xor(f, true))
@test @inferred(xor(f, f)) === f
@test @inferred(xor(f, t)) === t
@test @inferred(xor(t, f)) === t
@test @inferred(xor(t, t)) === f
@test @inferred(|(true, f))
@test @inferred(|(true, t)) === t
@test @inferred(|(f, true))
@test @inferred(|(t, true)) === t
@test @inferred(|(f, f)) === f
@test @inferred(|(f, t)) === t
@test @inferred(|(t, f)) === t
@test @inferred(|(t, t)) === t
@test @inferred(Base.:(&)(true, f)) === f
@test @inferred(Base.:(&)(true, t))
@test @inferred(Base.:(&)(f, true)) === f
@test @inferred(Base.:(&)(t, true))
@test @inferred(Base.:(&)(f, f)) === f
@test @inferred(Base.:(&)(f, t)) === f
@test @inferred(Base.:(&)(t, f)) === f
@test @inferred(Base.:(&)(t, t)) === t
@test @inferred(<(f, f)) === false
@test @inferred(<(f, t)) === true
@test @inferred(<(t, f)) === false
@test @inferred(<(t, t)) === false
@test @inferred(<=(f, f)) === true
@test @inferred(<=(f, t)) === true
@test @inferred(<=(t, f)) === false
@test @inferred(<=(t, t)) === true
@test @inferred(==(f, f)) === true
@test @inferred(==(f, t)) === false
@test @inferred(==(t, f)) === false
@test @inferred(==(t, t)) === true
@test @inferred(*(f, t)) === t & f
@test @inferred(-(f, t)) === StaticInt(f) - StaticInt(t)
@test @inferred(+(f, t)) === StaticInt(f) + StaticInt(t)
@test @inferred(^(t, f)) == ^(true, false)
@test @inferred(^(t, t)) == ^(true, true)
@test @inferred(^(2, f)) == 1
@test @inferred(^(2, t)) == 2
@test @inferred(^(BigInt(2), f)) == 1
@test @inferred(^(BigInt(2), t)) == 2
@test @inferred(div(t, t)) === t
@test_throws DivideError div(t, f)
@test @inferred(rem(t, t)) === f
@test_throws DivideError rem(t, f)
@test @inferred(mod(t, t)) === f
@test @inferred(all((t, t, t)))
@test !@inferred(all((t, f, t)))
@test !@inferred(all((f, f, f)))
@test @inferred(any((t, t, t)))
@test @inferred(any((t, f, t)))
@test !@inferred(any((f, f, f)))
x = StaticInt(1)
y = StaticInt(0)
z = StaticInt(-1)
@test @inferred(Static.eq(y)(x)) === f
@test @inferred(Static.eq(x, x)) === t
@test @inferred(Static.ne(y)(x)) === t
@test @inferred(Static.ne(x, x)) === f
@test @inferred(Static.gt(y)(x)) === t
@test @inferred(Static.gt(y, x)) === f
@test @inferred(Static.ge(y)(x)) === t
@test @inferred(Static.ge(y, x)) === f
@test @inferred(Static.lt(x)(y)) === t
@test @inferred(Static.lt(x, y)) === f
@test @inferred(Static.le(x)(y)) === t
@test @inferred(Static.le(x, y)) === f
@test @inferred(Static.ifelse(t, x, y)) === x
@test @inferred(Static.ifelse(f, x, y)) === y
@test @inferred(promote_rule(True, True)) <: StaticBool
@test @inferred(promote_rule(True, Bool)) <: Bool
@test @inferred(promote_rule(Bool, True)) <: Bool
end
@testset "StaticSymbol" begin
x = StaticSymbol(:x)
y = StaticSymbol("y")
z = StaticSymbol(1)
@test y === StaticSymbol(:y)
@test z === StaticSymbol(Symbol(1))
@test @inferred(StaticSymbol(x)) === x
@test @inferred(StaticSymbol(x, y)) === StaticSymbol(:x, :y)
@test @inferred(StaticSymbol(x, y, z)) === static(:xy1)
end
@testset "static interface" begin
v = Val((:a, 1, true))
@test static(1) === StaticInt(1)
@test static(true) === True()
@test static(:a) === StaticSymbol{:a}()
@test Symbol(static(:a)) === :a
@test static((:a, 1, true)) === (static(:a), static(1), static(true))
@test @inferred(static(v)) === (static(:a), static(1), static(true))
@test_throws ErrorException static("a")
@test @inferred(Static.is_static(v)) === True()
@test @inferred(Static.is_static(typeof(v))) === True()
@test @inferred(Static.is_static(typeof(static(true)))) === True()
@test @inferred(Static.is_static(typeof(static(1)))) === True()
@test @inferred(Static.is_static(typeof(static(:x)))) === True()
@test @inferred(Static.is_static(typeof(1))) === False()
@test @inferred(Static.is_static(typeof((static(:x),static(:x))))) === True()
@test @inferred(Static.is_static(typeof((static(:x),:x)))) === False()
@test @inferred(Static.is_static(typeof(static(1.0)))) === True()
@test @inferred(Static.known(v)) === (:a, 1, true)
@test @inferred(Static.known(typeof(v))) === (:a, 1, true)
@test @inferred(Static.known(typeof(static(true))))
@test @inferred(Static.known(typeof(static(false)))) === false
@test @inferred(Static.known(typeof(static(1.0)))) === 1.0
@test @inferred(Static.known(typeof(static(1)))) === 1
@test @inferred(Static.known(typeof(static(:x)))) === :x
@test @inferred(Static.known(typeof(1))) === missing
@test @inferred(Static.known(typeof((static(:x),static(:x))))) === (:x, :x)
@test @inferred(Static.known(typeof((static(:x),:x)))) === (:x, missing)
@test @inferred(Static.dynamic((static(:a), static(1), true))) === (:a, 1, true)
end
@testset "tuple utilities" begin
x = (static(1), static(2), static(3))
y = (static(3), static(2), static(1))
z = (static(1), static(2), static(3), static(4))
T = Tuple{Int,Float64,String}
@test @inferred(Static.invariant_permutation(x, x)) === True()
@test @inferred(Static.invariant_permutation(x, y)) === False()
@test @inferred(Static.invariant_permutation(x, z)) === False()
@test @inferred(Static.permute(x, Val(x))) === x
@test @inferred(Static.permute(x, (static(1), static(2)))) === (static(1), static(2))
@test @inferred(Static.permute(x, x)) === x
@test @inferred(Static.permute(x, y)) === y
@test @inferred(Static.eachop(getindex, x)) === x
get_tuple_add(::Type{T}, ::Type{X}, dim::StaticInt) where {T,X} = Tuple{Static._get_tuple(T, dim),X}
@test @inferred(Static.eachop_tuple(Static._get_tuple, y, T)) === Tuple{String,Float64,Int}
@test @inferred(Static.eachop_tuple(get_tuple_add, y, T, String)) === Tuple{Tuple{String,String},Tuple{Float64,String},Tuple{Int,String}}
@test @inferred(Static.find_first_eq(static(1), y)) === static(3)
# inferred is Union{Int,Nothing}
@test Static.find_first_eq(1, map(Int, y)) === 3
end
@testset "NDIndex" begin
x = NDIndex((1,2,3))
y = NDIndex((1,static(2),3))
z = NDIndex(static(3), static(3), static(3))
@testset "constructors" begin
@test static(CartesianIndex(3, 3, 3)) === z == Base.setindex(Base.setindex(x, 3, 1), 3, 2)
@test @inferred(CartesianIndex(z)) === @inferred(Static.dynamic(z)) === CartesianIndex(3, 3, 3)
@test @inferred(Static.known(z)) === (3, 3, 3)
@test Tuple(@inferred(NDIndex{0}())) === ()
@test @inferred(NDIndex{3}(1, static(2), 3)) === y
@test @inferred(NDIndex{3}((1, static(2), 3))) === y
@test @inferred(NDIndex{3}((1, static(2)))) === NDIndex(1, static(2), static(1))
@test @inferred(NDIndex(x, y)) === NDIndex(1, 2, 3, 1, static(2), 3)
end
@test @inferred(Base.IteratorsMD.split(x, Val(2))) === (NDIndex(1, 2), NDIndex(3,))
@test @inferred(length(x)) === 3
@test @inferred(length(typeof(x))) === 3
@test @inferred(y[2]) === 2
@test @inferred(y[static(2)]) === static(2)
@test @inferred(-y) === NDIndex((-1,-static(2),-3))
@test @inferred(y + y) === NDIndex((2,static(4),6))
@test @inferred(y - y) === NDIndex((0,static(0),0))
@test @inferred(zero(x)) === NDIndex(static(0),static(0),static(0))
@test @inferred(oneunit(x)) === NDIndex(static(1),static(1),static(1))
@test @inferred(x * 3) === NDIndex((3,6,9))
@test @inferred(3 * x) === NDIndex((3,6,9))
@test @inferred(min(x, z)) === x
@test @inferred(max(x, z)) === NDIndex(3, 3, 3)
@test !@inferred(isless(y, x))
@test @inferred(isless(x, z))
@test @inferred(Static.lt(oneunit(z), z)) === static(true)
A = rand(3,3,3);
@test @inferred(to_indices(A, axes(A), (x,))) === (1, 2, 3)
@test @inferred(to_indices(A, axes(A), ([y,y],))) == ([y, y],)
end
@test repr(static(float(1))) == "static($(float(1)))"
@test repr(static(1)) == "static(1)"
@test repr(static(:x)) == "static(:x)"
@test repr(static(true)) == "static(true)"
@test repr(static(CartesianIndex(1,1))) == "NDIndex(static(1), static(1))"
end
# for some reason this can't be inferred when in the "Static.jl" test set
known_length(x) = known_length(typeof(x))
known_length(::Type{T}) where {N,T<:Tuple{Vararg{Any,N}}} = N
known_length(::Type{T}) where {T} = missing
maybe_static_length(x) = Static.maybe_static(known_length, length, x)
x = ntuple(+, 10)
y = 1:10
@test @inferred(maybe_static_length(x)) === StaticInt(10)
@test @inferred(maybe_static_length(y)) === 10
include("float.jl")
#=
A = rand(3,4);
offset1(x::Base.OneTo) = static(1)
offset1(x::AbstractUnitRange) = first(x)
offsets(x) = Static._eachop(offset1, (axes(x),), Static.nstatic(Val(ndims(x))))
=#
| [
3500,
36125,
11,
24838,
198,
3500,
6208,
198,
198,
31,
9288,
2617,
366,
45442,
13,
20362,
1,
2221,
198,
220,
220,
220,
24838,
13,
9288,
62,
439,
7,
45442,
8,
628,
220,
220,
220,
2488,
9288,
2617,
366,
45442,
5317,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
9037,
7,
52,
5317,
7,
23,
4008,
24844,
36125,
5317,
7,
52,
5317,
7,
23,
4008,
24844,
36125,
5317,
90,
23,
92,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
318,
22570,
7,
45442,
5317,
7,
15,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
5145,
271,
22570,
7,
45442,
5317,
7,
16,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
5145,
271,
505,
7,
45442,
5317,
7,
15,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
318,
505,
7,
45442,
5317,
7,
16,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
505,
7,
45442,
5317,
7,
16,
22305,
24844,
36125,
5317,
7,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
22570,
7,
45442,
5317,
7,
16,
22305,
24844,
36125,
5317,
7,
15,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
505,
7,
45442,
5317,
4008,
24844,
36125,
5317,
7,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
22570,
7,
45442,
5317,
4008,
24844,
36125,
5317,
7,
15,
8,
24844,
36125,
5317,
7,
45442,
5317,
7,
7762,
7,
15,
22305,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
1288,
4906,
7,
505,
7,
45442,
5317,
4008,
1279,
25,
2558,
628,
220,
220,
220,
220,
220,
220,
220,
2124,
796,
36125,
5317,
7,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
33,
970,
7,
87,
4008,
318,
64,
347,
970,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
12804,
5317,
7,
87,
4008,
318,
64,
4403,
5317,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
46541,
7,
87,
4008,
24844,
2124,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
4,
7,
87,
11,
34142,
4008,
24844,
352,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
1332,
329,
18203,
84,
871,
290,
29409,
198,
220,
220,
220,
220,
220,
220,
220,
329,
1312,
18872,
230,
4377,
58,
45442,
5317,
7,
15,
828,
36125,
5317,
7,
16,
828,
36125,
5317,
7,
17,
828,
513,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
329,
474,
18872,
230,
4377,
58,
45442,
5317,
7,
15,
828,
36125,
5317,
7,
16,
828,
36125,
5317,
7,
17,
828,
513,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1312,
24844,
474,
24844,
513,
11405,
2555,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
329,
277,
18872,
230,
26076,
11,
532,
11,
1635,
11,
6184,
115,
11,
4064,
11,
9959,
11,
9609,
11,
13163,
11,
1222,
11,
930,
11,
2343,
232,
119,
11,
6624,
11,
41305,
11,
26870,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
357,
271,
22570,
7,
73,
8,
11405,
14808,
69,
24844,
6184,
115,
8,
8614,
357,
69,
24844,
4064,
22305,
11405,
2555,
1303,
18253,
7297,
4049,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
10385,
7,
5317,
11,
2488,
259,
18186,
7,
69,
7,
72,
11,
73,
22305,
6624,
277,
7,
1102,
1851,
7,
5317,
11,
1312,
828,
10385,
7,
5317,
11,
474,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1312,
6624,
513,
11405,
2270,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
329,
277,
18872,
230,
26076,
11,
532,
11,
1635,
11,
1220,
11,
6184,
115,
11,
4064,
11,
6624,
11,
41305,
11,
26870,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
266,
796,
277,
7,
1102,
1851,
7,
5317,
11,
1312,
828,
352,
13,
19,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
796,
277,
7,
16,
13,
19,
11,
10385,
7,
5317,
11,
1312,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
10385,
7,
4906,
1659,
7,
86,
828,
2488,
259,
18186,
7,
69,
7,
72,
11,
352,
13,
19,
22305,
24844,
266,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
10385,
7,
4906,
1659,
7,
87,
828,
2488,
259,
18186,
7,
69,
7,
16,
13,
19,
11,
1312,
22305,
24844,
2124,
1303,
611,
277,
318,
7297,
290,
1312,
24844,
36125,
5317,
7,
15,
828,
5860,
4600,
26705,
45,
63,
26,
12891,
779,
286,
796,
18604,
287,
2198,
13,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
14808,
7,
69,
24844,
6184,
115,
8,
8614,
357,
69,
24844,
4064,
4008,
11405,
357,
72,
24844,
36125,
5317,
7,
15,
22305,
11405,
2555,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
331,
796,
277,
7,
1102,
1851,
7,
5317,
11,
1312,
828,
362,
3373,
767,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1976,
796,
277,
7,
17,
3373,
767,
11,
10385,
7,
5317,
11,
1312,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
10385,
7,
4906,
1659,
7,
88,
828,
2488,
259,
18186,
7,
69,
7,
72,
11,
362,
3373,
767,
22305,
24844,
331,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
10385,
7,
4906,
1659,
7,
89,
828,
2488,
259,
18186,
7,
69,
7,
17,
3373,
767,
11,
1312,
22305,
24844,
1976,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
11801,
17257,
90,
5317,
1433,
92,
7,
45442,
5317,
32590,
24,
828,
1596,
8,
24844,
2558,
1433,
32590,
24,
2599,
5317,
1433,
7,
1558,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
11801,
17257,
90,
5317,
1433,
92,
32590,
22,
11,
36125,
5317,
7,
1129,
4008,
24844,
2558,
1433,
32590,
22,
2599,
5317,
1433,
7,
1129,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
11801,
17257,
32590,
1157,
11,
36125,
5317,
7,
1314,
4008,
24844,
532,
1157,
25,
1314,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
11801,
17257,
7,
45442,
5317,
32590,
1157,
828,
1315,
8,
24844,
532,
1157,
25,
1314,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
11801,
17257,
90,
5317,
92,
7,
45442,
5317,
32590,
1157,
828,
36125,
5317,
7,
1314,
4008,
24844,
532,
1157,
25,
1314,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
11801,
17257,
7,
45442,
5317,
32590,
1157,
828,
36125,
5317,
7,
1314,
4008,
24844,
532,
1157,
25,
1314,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
12178,
7,
45442,
5317,
7,
23,
4008,
24844,
9037,
7,
23,
13,
15,
8,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
1332,
2176,
7719,
3173,
284,
4155,
356,
836,
470,
2728,
18203,
84,
871,
198,
220,
220,
220,
220,
220,
220,
220,
25861,
796,
36125,
5317,
90,
16,
92,
198,
220,
220,
220,
220,
220,
220,
220,
14826,
796,
2099,
1659,
7,
16,
1003,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
30434,
796,
2099,
1659,
7,
14415,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
1102,
1851,
7,
11584,
11,
25861,
3419,
4008,
24844,
25861,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
11584,
11,
30434,
4008,
1279,
25,
7719,
62,
4906,
7,
5317,
11,
30434,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
11584,
11,
14826,
4008,
1279,
25,
7719,
62,
4906,
7,
5317,
11,
14826,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
11584,
11,
25861,
4008,
1279,
25,
2558,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
43730,
11,
25861,
4008,
1279,
25,
7719,
62,
4906,
7,
43730,
11,
2558,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
18465,
11,
25861,
4008,
1279,
25,
7719,
62,
4906,
7,
18465,
11,
2558,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
11584,
11,
25639,
4008,
1279,
25,
7719,
62,
4906,
7,
5317,
11,
25639,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
11584,
11,
10528,
4008,
1279,
25,
7719,
62,
4906,
7,
5317,
11,
10528,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
38176,
90,
43730,
11,
5317,
5512,
25861,
4008,
1279,
25,
7719,
62,
4906,
7,
38176,
90,
43730,
11,
5317,
5512,
2558,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
38176,
90,
18465,
11,
5317,
5512,
25861,
4008,
1279,
25,
7719,
62,
4906,
7,
38176,
90,
18465,
11,
5317,
5512,
2558,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
38176,
90,
18465,
11,
43730,
11,
5317,
5512,
25861,
4008,
1279,
25,
4479,
90,
18465,
11,
43730,
11,
5317,
92,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
38176,
90,
18465,
11,
43730,
5512,
25861,
4008,
1279,
25,
7719,
62,
4906,
7,
38176,
90,
18465,
11,
43730,
5512,
2558,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
11584,
11,
25639,
4008,
1279,
25,
7719,
62,
4906,
7,
5317,
11,
25639,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
14881,
13,
5080,
501,
6719,
16005,
90,
5317,
5512,
36125,
5317,
90,
16,
92,
4008,
1279,
25,
7308,
13,
5080,
501,
6719,
16005,
90,
5317,
92,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
9037,
7,
5317,
23,
32590,
1507,
4008,
24844,
9037,
32590,
1507,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
9037,
7,
15,
87,
891,
8,
24844,
9037,
7,
23516,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
9037,
7,
5317,
1433,
32590,
1507,
4008,
24844,
9037,
32590,
1507,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
9037,
7,
15,
47596,
891,
8,
24844,
9037,
7,
35916,
1129,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
39364,
7,
5317,
8,
6624,
807,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
9037,
7,
5317,
2624,
32590,
1507,
4008,
24844,
9037,
32590,
1507,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
9037,
7,
15,
87,
12927,
487,
891,
8,
24844,
9037,
7,
11785,
2920,
3134,
26050,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
45442,
33,
970,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
256,
796,
9037,
7,
12708,
7,
7942,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
277,
796,
36125,
33,
970,
7,
12708,
7,
9562,
4008,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
36125,
33,
970,
90,
7942,
92,
3419,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
36125,
33,
970,
90,
9562,
92,
3419,
24844,
277,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
5317,
7,
83,
4008,
24844,
36125,
5317,
7,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
5317,
7,
69,
4008,
24844,
36125,
5317,
7,
15,
8,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
93,
83,
8,
24844,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
93,
69,
8,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
0,
83,
8,
24844,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
0,
69,
8,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
10,
83,
8,
24844,
36125,
5317,
7,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
10,
69,
8,
24844,
36125,
5317,
7,
15,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
32590,
83,
8,
24844,
36125,
5317,
32590,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
32590,
69,
8,
24844,
36125,
5317,
7,
15,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
12683,
7,
83,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
8937,
7,
83,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
8937,
17,
7,
83,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
271,
22570,
7,
83,
4008,
24844,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
271,
505,
7,
83,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
271,
22570,
7,
69,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
271,
505,
7,
69,
4008,
24844,
277,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
87,
273,
7,
7942,
11,
277,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
87,
273,
7,
69,
11,
2081,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
87,
273,
7,
69,
11,
277,
4008,
24844,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
87,
273,
7,
69,
11,
256,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
87,
273,
7,
83,
11,
277,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
87,
273,
7,
83,
11,
256,
4008,
24844,
277,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
91,
7,
7942,
11,
277,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
91,
7,
7942,
11,
256,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
91,
7,
69,
11,
2081,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
91,
7,
83,
11,
2081,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
91,
7,
69,
11,
277,
4008,
24844,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
91,
7,
69,
11,
256,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
91,
7,
83,
11,
277,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
91,
7,
83,
11,
256,
4008,
24844,
256,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
14881,
11207,
39434,
5769,
7942,
11,
277,
4008,
24844,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
14881,
11207,
39434,
5769,
7942,
11,
256,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
14881,
11207,
39434,
5769,
69,
11,
2081,
4008,
24844,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
14881,
11207,
39434,
5769,
83,
11,
2081,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
14881,
11207,
39434,
5769,
69,
11,
277,
4008,
24844,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
14881,
11207,
39434,
5769,
69,
11,
256,
4008,
24844,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
14881,
11207,
39434,
5769,
83,
11,
277,
4008,
24844,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
14881,
11207,
39434,
5769,
83,
11,
256,
4008,
24844,
256,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
27,
7,
69,
11,
277,
4008,
24844,
3991,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
27,
7,
69,
11,
256,
4008,
24844,
2081,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
27,
7,
83,
11,
277,
4008,
24844,
3991,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
27,
7,
83,
11,
256,
4008,
24844,
3991,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
27,
16193,
69,
11,
277,
4008,
24844,
2081,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
27,
16193,
69,
11,
256,
4008,
24844,
2081,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
27,
16193,
83,
11,
277,
4008,
24844,
3991,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
27,
16193,
83,
11,
256,
4008,
24844,
2081,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
855,
7,
69,
11,
277,
4008,
24844,
2081,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
855,
7,
69,
11,
256,
4008,
24844,
3991,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
855,
7,
83,
11,
277,
4008,
24844,
3991,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
855,
7,
83,
11,
256,
4008,
24844,
2081,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
46491,
7,
69,
11,
256,
4008,
24844,
256,
1222,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
30420,
69,
11,
256,
4008,
24844,
36125,
5317,
7,
69,
8,
532,
36125,
5317,
7,
83,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
33747,
69,
11,
256,
4008,
24844,
36125,
5317,
7,
69,
8,
1343,
36125,
5317,
7,
83,
8,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
61,
7,
83,
11,
277,
4008,
6624,
10563,
7,
7942,
11,
3991,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
61,
7,
83,
11,
256,
4008,
6624,
10563,
7,
7942,
11,
2081,
8,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
61,
7,
17,
11,
277,
4008,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
61,
7,
17,
11,
256,
4008,
6624,
362,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
61,
7,
12804,
5317,
7,
17,
828,
277,
4008,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
61,
7,
12804,
5317,
7,
17,
828,
256,
4008,
6624,
362,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
7146,
7,
83,
11,
256,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
62,
400,
8516,
46894,
12331,
2659,
7,
83,
11,
277,
8,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
2787,
7,
83,
11,
256,
4008,
24844,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
62,
400,
8516,
46894,
12331,
816,
7,
83,
11,
277,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
4666,
7,
83,
11,
256,
4008,
24844,
277,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
439,
19510,
83,
11,
256,
11,
256,
22305,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
5145,
31,
259,
18186,
7,
439,
19510,
83,
11,
277,
11,
256,
22305,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
5145,
31,
259,
18186,
7,
439,
19510,
69,
11,
277,
11,
277,
22305,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
1092,
19510,
83,
11,
256,
11,
256,
22305,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
1092,
19510,
83,
11,
277,
11,
256,
22305,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
5145,
31,
259,
18186,
7,
1092,
19510,
69,
11,
277,
11,
277,
22305,
628,
220,
220,
220,
220,
220,
220,
220,
2124,
796,
36125,
5317,
7,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
331,
796,
36125,
5317,
7,
15,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1976,
796,
36125,
5317,
32590,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
27363,
7,
88,
5769,
87,
4008,
24844,
277,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
27363,
7,
87,
11,
2124,
4008,
24844,
256,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
710,
7,
88,
5769,
87,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
710,
7,
87,
11,
2124,
4008,
24844,
277,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
13655,
7,
88,
5769,
87,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
13655,
7,
88,
11,
2124,
4008,
24844,
277,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
469,
7,
88,
5769,
87,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
469,
7,
88,
11,
2124,
4008,
24844,
277,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
2528,
7,
87,
5769,
88,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
2528,
7,
87,
11,
331,
4008,
24844,
277,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
293,
7,
87,
5769,
88,
4008,
24844,
256,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
293,
7,
87,
11,
331,
4008,
24844,
277,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
361,
17772,
7,
83,
11,
2124,
11,
331,
4008,
24844,
2124,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
361,
17772,
7,
69,
11,
2124,
11,
331,
4008,
24844,
331,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
17821,
11,
6407,
4008,
1279,
25,
36125,
33,
970,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
17821,
11,
347,
970,
4008,
1279,
25,
347,
970,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
16963,
1258,
62,
25135,
7,
33,
970,
11,
6407,
4008,
1279,
25,
347,
970,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
45442,
13940,
23650,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
796,
36125,
13940,
23650,
7,
25,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
331,
796,
36125,
13940,
23650,
7203,
88,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
1976,
796,
36125,
13940,
23650,
7,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
331,
24844,
36125,
13940,
23650,
7,
25,
88,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
1976,
24844,
36125,
13940,
23650,
7,
13940,
23650,
7,
16,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13940,
23650,
7,
87,
4008,
24844,
2124,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13940,
23650,
7,
87,
11,
331,
4008,
24844,
36125,
13940,
23650,
7,
25,
87,
11,
1058,
88,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13940,
23650,
7,
87,
11,
331,
11,
1976,
4008,
24844,
9037,
7,
25,
5431,
16,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
12708,
7071,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
410,
796,
3254,
19510,
25,
64,
11,
352,
11,
2081,
4008,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
9037,
7,
16,
8,
24844,
36125,
5317,
7,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
9037,
7,
7942,
8,
24844,
6407,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
9037,
7,
25,
64,
8,
24844,
36125,
13940,
23650,
90,
25,
64,
92,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
38357,
7,
12708,
7,
25,
64,
4008,
24844,
1058,
64,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
9037,
19510,
25,
64,
11,
352,
11,
2081,
4008,
24844,
357,
12708,
7,
25,
64,
828,
9037,
7,
16,
828,
9037,
7,
7942,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
12708,
7,
85,
4008,
24844,
357,
12708,
7,
25,
64,
828,
9037,
7,
16,
828,
9037,
7,
7942,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
62,
400,
8516,
13047,
16922,
9037,
7203,
64,
4943,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
271,
62,
12708,
7,
85,
4008,
24844,
6407,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
271,
62,
12708,
7,
4906,
1659,
7,
85,
22305,
24844,
6407,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
271,
62,
12708,
7,
4906,
1659,
7,
12708,
7,
7942,
35514,
24844,
6407,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
271,
62,
12708,
7,
4906,
1659,
7,
12708,
7,
16,
35514,
24844,
6407,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
271,
62,
12708,
7,
4906,
1659,
7,
12708,
7,
25,
87,
35514,
24844,
6407,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
271,
62,
12708,
7,
4906,
1659,
7,
16,
22305,
24844,
10352,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
271,
62,
12708,
7,
4906,
1659,
19510,
12708,
7,
25,
87,
828,
12708,
7,
25,
87,
4008,
22305,
24844,
6407,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
271,
62,
12708,
7,
4906,
1659,
19510,
12708,
7,
25,
87,
828,
25,
87,
35514,
24844,
10352,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
271,
62,
12708,
7,
4906,
1659,
7,
12708,
7,
16,
13,
15,
35514,
24844,
6407,
3419,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
4002,
7,
85,
4008,
24844,
357,
25,
64,
11,
352,
11,
2081,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
4002,
7,
4906,
1659,
7,
85,
22305,
24844,
357,
25,
64,
11,
352,
11,
2081,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
4002,
7,
4906,
1659,
7,
12708,
7,
7942,
35514,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
4002,
7,
4906,
1659,
7,
12708,
7,
9562,
35514,
24844,
3991,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
4002,
7,
4906,
1659,
7,
12708,
7,
16,
13,
15,
35514,
24844,
352,
13,
15,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
4002,
7,
4906,
1659,
7,
12708,
7,
16,
35514,
24844,
352,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
4002,
7,
4906,
1659,
7,
12708,
7,
25,
87,
35514,
24844,
1058,
87,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
4002,
7,
4906,
1659,
7,
16,
22305,
24844,
4814,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
4002,
7,
4906,
1659,
19510,
12708,
7,
25,
87,
828,
12708,
7,
25,
87,
4008,
22305,
24844,
357,
25,
87,
11,
1058,
87,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
4002,
7,
4906,
1659,
19510,
12708,
7,
25,
87,
828,
25,
87,
35514,
24844,
357,
25,
87,
11,
4814,
8,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
67,
28995,
19510,
12708,
7,
25,
64,
828,
9037,
7,
16,
828,
2081,
22305,
24844,
357,
25,
64,
11,
352,
11,
2081,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
83,
29291,
20081,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
796,
357,
12708,
7,
16,
828,
9037,
7,
17,
828,
9037,
7,
18,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
331,
796,
357,
12708,
7,
18,
828,
9037,
7,
17,
828,
9037,
7,
16,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
1976,
796,
357,
12708,
7,
16,
828,
9037,
7,
17,
828,
9037,
7,
18,
828,
9037,
7,
19,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
309,
796,
309,
29291,
90,
5317,
11,
43879,
2414,
11,
10100,
92,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
16340,
2743,
415,
62,
16321,
7094,
7,
87,
11,
2124,
4008,
24844,
6407,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
16340,
2743,
415,
62,
16321,
7094,
7,
87,
11,
331,
4008,
24844,
10352,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
16340,
2743,
415,
62,
16321,
7094,
7,
87,
11,
1976,
4008,
24844,
10352,
3419,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
16321,
1133,
7,
87,
11,
3254,
7,
87,
22305,
24844,
2124,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
16321,
1133,
7,
87,
11,
357,
12708,
7,
16,
828,
9037,
7,
17,
35514,
24844,
357,
12708,
7,
16,
828,
9037,
7,
17,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
16321,
1133,
7,
87,
11,
2124,
4008,
24844,
2124,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
16321,
1133,
7,
87,
11,
331,
4008,
24844,
331,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
27379,
404,
7,
1136,
9630,
11,
2124,
4008,
24844,
2124,
628,
220,
220,
220,
220,
220,
220,
220,
651,
62,
83,
29291,
62,
2860,
7,
3712,
6030,
90,
51,
5512,
7904,
6030,
90,
55,
5512,
5391,
3712,
45442,
5317,
8,
810,
1391,
51,
11,
55,
92,
796,
309,
29291,
90,
45442,
13557,
1136,
62,
83,
29291,
7,
51,
11,
5391,
828,
55,
92,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
27379,
404,
62,
83,
29291,
7,
45442,
13557,
1136,
62,
83,
29291,
11,
331,
11,
309,
4008,
24844,
309,
29291,
90,
10100,
11,
43879,
2414,
11,
5317,
92,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
27379,
404,
62,
83,
29291,
7,
1136,
62,
83,
29291,
62,
2860,
11,
331,
11,
309,
11,
10903,
4008,
24844,
309,
29291,
90,
51,
29291,
90,
10100,
11,
10100,
5512,
51,
29291,
90,
43879,
2414,
11,
10100,
5512,
51,
29291,
90,
5317,
11,
10100,
11709,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
19796,
62,
11085,
62,
27363,
7,
12708,
7,
16,
828,
331,
4008,
24844,
9037,
7,
18,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
41240,
318,
4479,
90,
5317,
11,
18465,
92,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
36125,
13,
19796,
62,
11085,
62,
27363,
7,
16,
11,
3975,
7,
5317,
11,
331,
4008,
24844,
513,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
2617,
366,
8575,
15732,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
796,
25524,
15732,
19510,
16,
11,
17,
11,
18,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
331,
796,
25524,
15732,
19510,
16,
11,
12708,
7,
17,
828,
18,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
1976,
796,
25524,
15732,
7,
12708,
7,
18,
828,
9037,
7,
18,
828,
9037,
7,
18,
4008,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2617,
366,
41571,
669,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
9037,
7,
43476,
35610,
15732,
7,
18,
11,
513,
11,
513,
4008,
24844,
1976,
6624,
7308,
13,
2617,
9630,
7,
14881,
13,
2617,
9630,
7,
87,
11,
513,
11,
352,
828,
513,
11,
362,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
43476,
35610,
15732,
7,
89,
4008,
24844,
2488,
259,
18186,
7,
45442,
13,
67,
28995,
7,
89,
4008,
24844,
13690,
35610,
15732,
7,
18,
11,
513,
11,
513,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
4002,
7,
89,
4008,
24844,
357,
18,
11,
513,
11,
513,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
309,
29291,
7,
31,
259,
18186,
7,
8575,
15732,
90,
15,
92,
3419,
4008,
24844,
7499,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
8575,
15732,
90,
18,
92,
7,
16,
11,
9037,
7,
17,
828,
513,
4008,
24844,
331,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
8575,
15732,
90,
18,
92,
19510,
16,
11,
9037,
7,
17,
828,
513,
22305,
24844,
331,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
8575,
15732,
90,
18,
92,
19510,
16,
11,
9037,
7,
17,
35514,
24844,
25524,
15732,
7,
16,
11,
9037,
7,
17,
828,
9037,
7,
16,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
8575,
15732,
7,
87,
11,
331,
4008,
24844,
25524,
15732,
7,
16,
11,
362,
11,
513,
11,
352,
11,
9037,
7,
17,
828,
513,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
14881,
13,
29993,
2024,
12740,
13,
35312,
7,
87,
11,
3254,
7,
17,
22305,
24844,
357,
8575,
15732,
7,
16,
11,
362,
828,
25524,
15732,
7,
18,
11,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
13664,
7,
87,
4008,
24844,
513,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
13664,
7,
4906,
1659,
7,
87,
22305,
24844,
513,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
88,
58,
17,
12962,
24844,
362,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
88,
58,
12708,
7,
17,
8,
12962,
24844,
9037,
7,
17,
8,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
32590,
88,
8,
24844,
25524,
15732,
19510,
12,
16,
12095,
12708,
7,
17,
828,
12,
18,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
88,
1343,
331,
8,
24844,
25524,
15732,
19510,
17,
11,
12708,
7,
19,
828,
21,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
88,
532,
331,
8,
24844,
25524,
15732,
19510,
15,
11,
12708,
7,
15,
828,
15,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
22570,
7,
87,
4008,
24844,
25524,
15732,
7,
12708,
7,
15,
828,
12708,
7,
15,
828,
12708,
7,
15,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
505,
20850,
7,
87,
4008,
24844,
25524,
15732,
7,
12708,
7,
16,
828,
12708,
7,
16,
828,
12708,
7,
16,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
87,
1635,
513,
8,
24844,
25524,
15732,
19510,
18,
11,
21,
11,
24,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
18,
1635,
2124,
8,
24844,
25524,
15732,
19510,
18,
11,
21,
11,
24,
4008,
628,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
1084,
7,
87,
11,
1976,
4008,
24844,
2124,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
9806,
7,
87,
11,
1976,
4008,
24844,
25524,
15732,
7,
18,
11,
513,
11,
513,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
5145,
31,
259,
18186,
7,
271,
1203,
7,
88,
11,
2124,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
271,
1203,
7,
87,
11,
1976,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
45442,
13,
2528,
7,
505,
20850,
7,
89,
828,
1976,
4008,
24844,
9037,
7,
7942,
8,
628,
220,
220,
220,
220,
220,
220,
220,
317,
796,
43720,
7,
18,
11,
18,
11,
18,
1776,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
1462,
62,
521,
1063,
7,
32,
11,
34197,
7,
32,
828,
357,
87,
11,
22305,
24844,
357,
16,
11,
362,
11,
513,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2488,
259,
18186,
7,
1462,
62,
521,
1063,
7,
32,
11,
34197,
7,
32,
828,
29565,
88,
11,
88,
4357,
22305,
6624,
29565,
88,
11,
331,
4357,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2488,
9288,
41575,
7,
12708,
7,
22468,
7,
16,
22305,
6624,
366,
12708,
16763,
7,
22468,
7,
16,
4008,
16725,
198,
220,
220,
220,
2488,
9288,
41575,
7,
12708,
7,
16,
4008,
6624,
366,
12708,
7,
16,
16725,
198,
220,
220,
220,
2488,
9288,
41575,
7,
12708,
7,
25,
87,
4008,
6624,
366,
12708,
7,
25,
87,
16725,
198,
220,
220,
220,
2488,
9288,
41575,
7,
12708,
7,
7942,
4008,
6624,
366,
12708,
7,
7942,
16725,
198,
220,
220,
220,
2488,
9288,
41575,
7,
12708,
7,
43476,
35610,
15732,
7,
16,
11,
16,
22305,
6624,
366,
8575,
15732,
7,
12708,
7,
16,
828,
9037,
7,
16,
4008,
1,
198,
437,
198,
198,
2,
329,
617,
1738,
428,
460,
470,
307,
41240,
618,
287,
262,
366,
45442,
13,
20362,
1,
1332,
900,
198,
4002,
62,
13664,
7,
87,
8,
796,
1900,
62,
13664,
7,
4906,
1659,
7,
87,
4008,
198,
4002,
62,
13664,
7,
3712,
6030,
90,
51,
30072,
810,
1391,
45,
11,
51,
27,
25,
51,
29291,
90,
19852,
853,
90,
7149,
11,
45,
42535,
796,
399,
198,
4002,
62,
13664,
7,
3712,
6030,
90,
51,
30072,
810,
1391,
51,
92,
796,
4814,
198,
25991,
62,
12708,
62,
13664,
7,
87,
8,
796,
36125,
13,
25991,
62,
12708,
7,
4002,
62,
13664,
11,
4129,
11,
2124,
8,
198,
87,
796,
299,
83,
29291,
7,
28200,
838,
8,
198,
88,
796,
352,
25,
940,
198,
31,
9288,
2488,
259,
18186,
7,
25991,
62,
12708,
62,
13664,
7,
87,
4008,
24844,
36125,
5317,
7,
940,
8,
198,
31,
9288,
2488,
259,
18186,
7,
25991,
62,
12708,
62,
13664,
7,
88,
4008,
24844,
838,
198,
198,
17256,
7203,
22468,
13,
20362,
4943,
628,
198,
2,
28,
198,
32,
796,
43720,
7,
18,
11,
19,
1776,
198,
198,
28968,
16,
7,
87,
3712,
14881,
13,
3198,
2514,
8,
796,
9037,
7,
16,
8,
198,
28968,
16,
7,
87,
3712,
23839,
26453,
17257,
8,
796,
717,
7,
87,
8,
198,
198,
8210,
1039,
7,
87,
8,
796,
36125,
13557,
27379,
404,
7,
28968,
16,
11,
357,
897,
274,
7,
87,
828,
828,
36125,
13,
77,
12708,
7,
7762,
7,
358,
12078,
7,
87,
35514,
198,
198,
46249,
198
] | 2.079461 | 7,123 |
using JLD, Images
function main(dim)
points = load("./out/20k.jld", "points")
img = zeros(RGB,dim,dim)
for c in points
z = c
while abs(z) < 2
x = trunc(Int,(real(z) + 2)*dim/3); y = trunc(Int,(imag(z) + 1.5)*dim/3)
0<x<dim>y>0 && (img[x,y] += RGB(0.0005,0,0))
z = z^2 + c
end
end
return map(clamp01nan, img)
end
@time save("./images/t1.png", main(1500)) | [
3500,
449,
11163,
11,
5382,
201,
198,
201,
198,
8818,
1388,
7,
27740,
8,
201,
198,
220,
220,
220,
2173,
796,
3440,
7,
1911,
14,
448,
14,
1238,
74,
13,
73,
335,
1600,
366,
13033,
4943,
201,
198,
220,
220,
220,
33705,
796,
1976,
27498,
7,
36982,
11,
27740,
11,
27740,
8,
201,
198,
220,
220,
220,
329,
269,
287,
2173,
201,
198,
220,
220,
220,
220,
220,
220,
220,
1976,
796,
269,
201,
198,
220,
220,
220,
220,
220,
220,
220,
981,
2352,
7,
89,
8,
1279,
362,
220,
201,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
796,
40122,
7,
5317,
11,
7,
5305,
7,
89,
8,
1343,
362,
27493,
27740,
14,
18,
1776,
331,
796,
40122,
7,
5317,
11,
7,
48466,
7,
89,
8,
1343,
352,
13,
20,
27493,
27740,
14,
18,
8,
201,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
657,
27,
87,
27,
27740,
29,
88,
29,
15,
11405,
357,
9600,
58,
87,
11,
88,
60,
15853,
25228,
7,
15,
13,
830,
20,
11,
15,
11,
15,
4008,
201,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1976,
796,
1976,
61,
17,
1343,
269,
201,
198,
220,
220,
220,
220,
220,
220,
220,
886,
201,
198,
220,
220,
220,
886,
201,
198,
220,
220,
220,
1441,
3975,
7,
565,
696,
486,
12647,
11,
33705,
8,
201,
198,
437,
201,
198,
201,
198,
31,
2435,
3613,
7,
1911,
14,
17566,
14,
83,
16,
13,
11134,
1600,
1388,
7,
33698,
4008
] | 1.751938 | 258 |
clear()
@testset "check pooling op's gradient at single dim" begin
for d in [1 2 3]
for pool in [maximum minimum sum mean linearpool exppool]
@testset "check $pool op's gradient at dim = $d" begin
DIMS = d
TYPE = Array{Float64};
# [1] prepare input data and its label
inputdims = 64;
timeSteps = 16;
batchsize = 32;
x = Variable(rand(inputdims, timeSteps, batchsize); type=TYPE, keepsgrad=true,);
if d==1;l = Variable(rand(1, timeSteps, batchsize); type=TYPE);end
if d==2;l = Variable(rand(inputdims, 1, batchsize); type=TYPE);end
if d==3;l = Variable(rand(inputdims, timeSteps, 1); type=TYPE);end
# [2] forward and backward propagation
COST1 = cost(mseLoss(pool(x; dims=DIMS), l));
backward();
# [3] with a samll change of a weight
GRAD = x.delta[1];
DELTA = 1e-6;
x.value[1] += DELTA;
# [4] forward and backward propagation
COST2 = cost(mseLoss(pool(x; dims=DIMS), l));
backward();
# [5] check if the auto-grad is true or not
dLdW = (COST2 - COST1)/DELTA; # numerical gradient
err = abs((dLdW-GRAD)/(GRAD+eps(Float32)))*100; # relative error in %
err = err < 1e-1 ? 0.0 : err;
@test err<1.0
end
end
end
end
@testset "check pooling op's gradient at mutiple dims" begin
for pool in [maximum minimum sum mean linearpool exppool]
@testset "check $pool op's gradient" begin
DIMS = (1,2)
TYPE = Array{Float64};
# [1] prepare input data and its label
inputdims = 64;
timeSteps = 16;
batchsize = 32;
x = Variable(rand(inputdims, timeSteps, batchsize); type=TYPE,keepsgrad=true);
l = Variable(rand(1, 1, batchsize); type=TYPE);
# [2] forward and backward propagation
COST1 = cost(mseLoss(pool(x; dims=DIMS), l));
backward();
# [3] with a samll change of a weight
GRAD = x.delta[1];
DELTA = 1e-6;
x.value[1] += DELTA;
# [4] forward and backward propagation with a samll change of a weight
COST2 = cost(mseLoss(pool(x; dims=DIMS), l));
backward();
# [5] check if the auto-grad is true or not
dLdW = (COST2 - COST1)/DELTA; # numerical gradient
err = abs((dLdW-GRAD)/(GRAD+eps(Float64)))*100; # relative error in %
err = err < 1e-1 ? 0.0 : err;
@test err<1.0
end
end
end
@testset "check maxmin and minmax op's gradient at mutiple dims" begin
for pool in [maxmin minmax]
@testset "check $pool op's gradient" begin
DIM1 = 1
DIM2 = 2
TYPE = Array{Float64};
# [1] prepare input data and its label
inputdims = 64;
timeSteps = 16;
batchsize = 32;
x = Variable(rand(inputdims, timeSteps, batchsize); type=TYPE,keepsgrad=true);
l = Variable(rand(1, 1, batchsize); type=TYPE);
# [2] forward and backward propagation
COST1 = mseLoss(pool(x; dims1=DIM1, dims2=DIM2), l) |> cost
backward();
# [3] with a samll change of a weight
GRAD = x.delta[1];
DELTA = 1e-6;
x.value[1] += DELTA;
# [4] forward and backward propagation with a samll change of a weight
COST2 = mseLoss(pool(x; dims1=DIM1, dims2=DIM2), l) |> cost
backward();
# [5] check if the auto-grad is true or not
dLdW = (COST2 - COST1)/DELTA; # numerical gradient
err = abs((dLdW-GRAD)/(GRAD+eps(Float64)))*100; # relative error in %
err = err < 1e-1 ? 0.0 : err;
@test err<1.0
end
end
end
| [
20063,
3419,
198,
31,
9288,
2617,
366,
9122,
5933,
278,
1034,
338,
31312,
379,
2060,
5391,
1,
2221,
198,
220,
220,
220,
329,
288,
287,
685,
16,
362,
513,
60,
198,
220,
220,
220,
220,
220,
220,
220,
329,
5933,
287,
685,
47033,
5288,
2160,
1612,
14174,
7742,
409,
381,
970,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2617,
366,
9122,
720,
7742,
1034,
338,
31312,
379,
5391,
796,
720,
67,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
360,
3955,
50,
796,
288,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
41876,
796,
15690,
90,
43879,
2414,
19629,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
16,
60,
8335,
5128,
1366,
290,
663,
6167,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
5128,
67,
12078,
796,
5598,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
640,
8600,
82,
796,
1467,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
15458,
7857,
796,
3933,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
796,
35748,
7,
25192,
7,
15414,
67,
12078,
11,
640,
8600,
82,
11,
15458,
7857,
1776,
2099,
28,
25216,
11,
7622,
9744,
28,
7942,
11,
1776,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
288,
855,
16,
26,
75,
796,
35748,
7,
25192,
7,
16,
11,
640,
8600,
82,
11,
15458,
7857,
1776,
2099,
28,
25216,
1776,
437,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
288,
855,
17,
26,
75,
796,
35748,
7,
25192,
7,
15414,
67,
12078,
11,
352,
11,
15458,
7857,
1776,
2099,
28,
25216,
1776,
437,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
288,
855,
18,
26,
75,
796,
35748,
7,
25192,
7,
15414,
67,
12078,
11,
640,
8600,
82,
11,
352,
1776,
2099,
28,
25216,
1776,
437,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
17,
60,
2651,
290,
19528,
43594,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7375,
2257,
16,
796,
1575,
7,
76,
325,
43,
793,
7,
7742,
7,
87,
26,
5391,
82,
28,
35,
3955,
50,
828,
300,
18125,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
19528,
9783,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
18,
60,
351,
257,
6072,
297,
1487,
286,
257,
3463,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
10863,
2885,
796,
2124,
13,
67,
12514,
58,
16,
11208,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
28163,
5603,
796,
352,
68,
12,
21,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
13,
8367,
58,
16,
60,
15853,
28163,
5603,
26,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
19,
60,
2651,
290,
19528,
43594,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7375,
2257,
17,
796,
1575,
7,
76,
325,
43,
793,
7,
7742,
7,
87,
26,
5391,
82,
28,
35,
3955,
50,
828,
300,
18125,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
19528,
9783,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
20,
60,
2198,
611,
262,
8295,
12,
9744,
318,
2081,
393,
407,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
288,
43,
67,
54,
796,
357,
8220,
2257,
17,
532,
7375,
2257,
16,
20679,
35,
3698,
5603,
26,
220,
220,
1303,
29052,
31312,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
11454,
220,
796,
2352,
19510,
45582,
67,
54,
12,
10761,
2885,
20679,
7,
10761,
2885,
10,
25386,
7,
43879,
2624,
22305,
9,
3064,
26,
220,
1303,
3585,
4049,
287,
4064,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
11454,
220,
796,
11454,
1279,
352,
68,
12,
16,
5633,
657,
13,
15,
1058,
11454,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
11454,
27,
16,
13,
15,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
437,
628,
198,
31,
9288,
2617,
366,
9122,
5933,
278,
1034,
338,
31312,
379,
4517,
2480,
5391,
82,
1,
2221,
198,
220,
220,
220,
329,
5933,
287,
685,
47033,
5288,
2160,
1612,
14174,
7742,
409,
381,
970,
60,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2617,
366,
9122,
720,
7742,
1034,
338,
31312,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
360,
3955,
50,
796,
357,
16,
11,
17,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
41876,
796,
15690,
90,
43879,
2414,
19629,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
16,
60,
8335,
5128,
1366,
290,
663,
6167,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
5128,
67,
12078,
796,
5598,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
640,
8600,
82,
796,
1467,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
15458,
7857,
796,
3933,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
796,
35748,
7,
25192,
7,
15414,
67,
12078,
11,
640,
8600,
82,
11,
15458,
7857,
1776,
2099,
28,
25216,
11,
14894,
82,
9744,
28,
7942,
1776,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
300,
796,
35748,
7,
25192,
7,
16,
11,
220,
220,
220,
220,
220,
220,
220,
220,
352,
11,
220,
220,
220,
220,
220,
220,
220,
220,
15458,
7857,
1776,
2099,
28,
25216,
1776,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
17,
60,
2651,
290,
19528,
43594,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7375,
2257,
16,
796,
1575,
7,
76,
325,
43,
793,
7,
7742,
7,
87,
26,
5391,
82,
28,
35,
3955,
50,
828,
300,
18125,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
19528,
9783,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
18,
60,
351,
257,
6072,
297,
1487,
286,
257,
3463,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
10863,
2885,
796,
2124,
13,
67,
12514,
58,
16,
11208,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
28163,
5603,
796,
352,
68,
12,
21,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
13,
8367,
58,
16,
60,
15853,
28163,
5603,
26,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
19,
60,
2651,
290,
19528,
43594,
351,
257,
6072,
297,
1487,
286,
257,
3463,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7375,
2257,
17,
796,
1575,
7,
76,
325,
43,
793,
7,
7742,
7,
87,
26,
5391,
82,
28,
35,
3955,
50,
828,
300,
18125,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
19528,
9783,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
20,
60,
2198,
611,
262,
8295,
12,
9744,
318,
2081,
393,
407,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
288,
43,
67,
54,
796,
357,
8220,
2257,
17,
532,
7375,
2257,
16,
20679,
35,
3698,
5603,
26,
220,
220,
1303,
29052,
31312,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
11454,
220,
796,
2352,
19510,
45582,
67,
54,
12,
10761,
2885,
20679,
7,
10761,
2885,
10,
25386,
7,
43879,
2414,
22305,
9,
3064,
26,
220,
1303,
3585,
4049,
287,
4064,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
11454,
220,
796,
11454,
1279,
352,
68,
12,
16,
5633,
657,
13,
15,
1058,
11454,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
11454,
27,
16,
13,
15,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
437,
628,
198,
31,
9288,
2617,
366,
9122,
3509,
1084,
290,
949,
9806,
1034,
338,
31312,
379,
4517,
2480,
5391,
82,
1,
2221,
198,
220,
220,
220,
329,
5933,
287,
685,
9806,
1084,
949,
9806,
60,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
2617,
366,
9122,
720,
7742,
1034,
338,
31312,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
360,
3955,
16,
796,
352,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
360,
3955,
17,
796,
362,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
41876,
796,
15690,
90,
43879,
2414,
19629,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
16,
60,
8335,
5128,
1366,
290,
663,
6167,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
5128,
67,
12078,
796,
5598,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
640,
8600,
82,
796,
1467,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
15458,
7857,
796,
3933,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
796,
35748,
7,
25192,
7,
15414,
67,
12078,
11,
640,
8600,
82,
11,
15458,
7857,
1776,
2099,
28,
25216,
11,
14894,
82,
9744,
28,
7942,
1776,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
300,
796,
35748,
7,
25192,
7,
16,
11,
220,
220,
220,
220,
220,
220,
220,
220,
352,
11,
220,
220,
220,
220,
220,
220,
220,
220,
15458,
7857,
1776,
2099,
28,
25216,
1776,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
17,
60,
2651,
290,
19528,
43594,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7375,
2257,
16,
796,
285,
325,
43,
793,
7,
7742,
7,
87,
26,
5391,
82,
16,
28,
35,
3955,
16,
11,
5391,
82,
17,
28,
35,
3955,
17,
828,
300,
8,
930,
29,
1575,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
19528,
9783,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
18,
60,
351,
257,
6072,
297,
1487,
286,
257,
3463,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
10863,
2885,
796,
2124,
13,
67,
12514,
58,
16,
11208,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
28163,
5603,
796,
352,
68,
12,
21,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
13,
8367,
58,
16,
60,
15853,
28163,
5603,
26,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
19,
60,
2651,
290,
19528,
43594,
351,
257,
6072,
297,
1487,
286,
257,
3463,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
7375,
2257,
17,
796,
285,
325,
43,
793,
7,
7742,
7,
87,
26,
5391,
82,
16,
28,
35,
3955,
16,
11,
5391,
82,
17,
28,
35,
3955,
17,
828,
300,
8,
930,
29,
1575,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
19528,
9783,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
685,
20,
60,
2198,
611,
262,
8295,
12,
9744,
318,
2081,
393,
407,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
288,
43,
67,
54,
796,
357,
8220,
2257,
17,
532,
7375,
2257,
16,
20679,
35,
3698,
5603,
26,
220,
220,
1303,
29052,
31312,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
11454,
220,
796,
2352,
19510,
45582,
67,
54,
12,
10761,
2885,
20679,
7,
10761,
2885,
10,
25386,
7,
43879,
2414,
22305,
9,
3064,
26,
220,
1303,
3585,
4049,
287,
4064,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
11454,
220,
796,
11454,
1279,
352,
68,
12,
16,
5633,
657,
13,
15,
1058,
11454,
26,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
11454,
27,
16,
13,
15,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
437,
198
] | 1.887625 | 2,198 |
# ====================================================================
# Load Different Logger
# ====================================================================
"""
load_logger_from_config(file_path::AbstractString)::TeeLogger
Create a combined logger from a config file path.
### Note
A combined logger is a `TeeLogger` struct that allows to send the same log message to
all loggers included in the combined logger at once.
"""
function load_logger_from_config(file_path::AbstractString)
json_string = read(file_path, String)
json3_dict = JSON3.read(json_string) #, Dict
return load_logger_from_config(json3_dict)
end
"""
load_logger_from_config(config::Dict)::TeeLogger
Create a `TeeLoger`, a collection of logger, from a configuration `Dict`.
### Note
- A `TeeLogger` struct allows to send the same log message to all loggers included in the `TeeLoger` at once.
- The configuration `Dict` requires as `"logging"` field, see example for more details.
### Example
```julia
config = Dict(
...,
"logging" => [
# logger_type => logger_config_dict,
"SeqLogger" => Dict(...),
...
],
...
)
```
### Returns
`TeeLogger` as defined in `config`
"""
function load_logger_from_config(config::AbstractDict)
loggers = [
get_logger(string(logger_type), logger_specs) for (logger_type, logger_specs) in config["logging"]
]
return TeeLogger(loggers...)
end
# ====================================================================
# Load Single Logger
# ====================================================================
"""
load_seqlogger(logger_config::AbstractDict)::Union{SeqLogger, TransformerLogger}
Return a `SeqLogger` or `TransformerLogger` according to `logger_config`.
### Config Parameters
- `"server_url"` -- required
- `"min_level"` -- required (`"DEBUG", "INFO", "WARN", "ERROR"`)
- `"transformation"` -- optional, default `identity`
- `"api_key"` -- optional, default `""`
- `"batch_size"` -- optional, default `10`
All other config parameters are used as global event properties.
### Example
```julia
log_dict = Dict(
"server_url" => "http://subdn215:5341/",
"min_level" => "INFO",
"batch_size" => 12,
"App" => "SeqLoggers_Test",
"Env" => "Test"
)
seq_logger = SeqLoggers.load_seqlogger(log_dict)
```
"""
function load_seqlogger(logger_config::AbstractDict)
server_url = logger_config["server_url"]
min_level = logger_config["min_level"] |> get_log_level
transformation_str = get(logger_config, "transformation", "identity")
transformation = transformation_str |> get_transformation_function
# Create a NamedTuple from remaining Config Keyvalue Pairs
kwarg_keys = filter(
key -> string(key) ∉ ["server_url", "min_level", "transformation"], keys(logger_config)
)
kwarg_keys_names = Tuple(Symbol(key) for key in kwarg_keys)
kwarg_keys_values = [logger_config[key] for key in kwarg_keys]
kwarg_keys = NamedTuple{kwarg_keys_names}(kwarg_keys_values)
return SeqLogger(
server_url; min_level=min_level, kwarg_keys...
) |> transformation
end
"""
load_consolelogger(logger_config::AbstractDict)::Union{ConsoleLogger, TransformerLogger}
Return a `ConsoleLogger` or `TransformerLogger` according to `logger_config`.
### Config Parameters
- `"min_level"` -- required (`"DEBUG", "INFO", "WARN", "ERROR"`)
- `"transformation"` -- optional, default `identity`
### Example
```julia
logging_config = Dict(
"min_level" => "ERROR",
"transformation" => "add_timestamp",
)
seq_logger = SeqLoggers.load_consolelogger(log_dict)
```
"""
function load_consolelogger(logger_config::AbstractDict)
min_level = logger_config["min_level"] |> get_log_level
transformation_str = get(logger_config, "transformation", "identity")
transformation = transformation_str |> get_transformation_function
return ConsoleLogger(stderr, min_level) |> transformation
end
"""
load_consolelogger(logger_config::AbstractDict)::AbstractLogger
Return a `MinLevelLogger{FileLogger}` or `TransformerLogger` according to `logger_config`.
### Config Parameters
- `"file_path"` -- required
- `"min_level"` -- required (`"DEBUG", "INFO", "WARN", "ERROR"`)
- `"append"` -- optional, default `true`, append to file if `true`, otherwise truncate file. (See [`LoggingExtras.FileLogger`](@ref) for more information.)
- `"transformation"` -- optional, default `identity`
### Example
```julia
logging_config = Dict(
"file_path" => "C:/Temp/test.log",
"min_level" => "ERROR",
"append" => true,
"transformation" => "add_timestamp",
)
seq_logger = SeqLoggers.load_filelogger(log_dict)
```
"""
function load_filelogger(logger_config::AbstractDict)
min_level = logger_config["min_level"] |> get_log_level
file_path = logger_config["file_path"]
append = get(logger_config, "append", true)
transformation_str = get(logger_config, "transformation", "identity")
transformation = transformation_str |> get_transformation_function
return MinLevelLogger(FileLogger(file_path; append=append), min_level) |> transformation
end
"""
load_advanced_filelogger(logger_config::AbstractDict)::AbstractLogger
Return a `DatetimeRotatingFileLogger` or `TransformerLogger` according to `logger_config`.
### Config Parameters
- `"dir_path"` -- required
- `"min_level"` -- required (`"DEBUG", "INFO", "WARN", "ERROR"`)
- `"file_name_pattern"` -- required e.g. `"\\a\\c\\c\\e\\s\\s-YYYY-mm-dd-HH-MM.\\l\\o\\g"`
- `"transformation"` -- optional, default `identity`
### Example
```julia
logging_config = Dict(
"dir_path" => "C:/Temp",
"min_level" => "ERROR",
"file_name_pattern" => "\\a\\c\\c\\e\\s\\s-YYYY-mm-dd-HH-MM.\\l\\o\\g",
"transformation" => "add_timestamp",
)
seq_logger = SeqLoggers.load_advanced_filelogger(log_dict)
```
"""
function load_advanced_filelogger(logger_config::AbstractDict)
min_level = logger_config["min_level"] |> get_log_level
dir_path = logger_config["dir_path"]
file_name_pattern = logger_config["file_name_pattern"]
transformation_str = get(logger_config, "transformation", "identity")
transformation = transformation_str |> get_transformation_function
return AdvancedFileLogger(
dir_path,
file_name_pattern;
log_format_function=print_standard_format,
min_level=min_level
) |> transformation
end
# ====================================================================
# Logger Type Mapping and Register
# ====================================================================
"""
get_logger(logger_type::AbstractString, logger_config::AbstractDict)::AbstractLogger
Create logger struct from logger type name and `Dict` with required parameters.
By default, the following logger types are supported:
- `"SeqLogger"` → [`SeqLogger`](@ref)
- `"ConsoleLogger"` → [`ConsoleLogger`](@ref)
- `"FileLogger"` → [`FileLogger`](@ref)
Use [`register_logger!`](@ref) to add custom `AbstractLogger`s.
"""
function get_logger(logger_type::AbstractString, logger_config::AbstractDict)
logger_constructor = get(LOGGER_TYPE_MAPPING, logger_type, nothing)
if isnothing(logger_constructor)
throw(
ArgumentError(
"There is no logger corresponding to the key `$logger_type`. " *
"Available options are $(collect(keys(LOGGER_TYPE_MAPPING))). " *
"Use `register_logger!` to add new logger types."
)
)
end
return logger_constructor(logger_config)
end
"""
register_logger!(logger_type::AbstractString, logger_constructor::Function)
Register a new logger type.
Registering enables the user to use custom `AbstractLogger` struct, defined outside of `SeqLoggers`,
to be used with [`load_logger_from_config`](@ref).
"""
function register_logger!(logger_type::AbstractString, logger_constructor::Function)
if haskey(LOGGER_TYPE_MAPPING, logger_type)
@warn "Logger type `$logger_type` already exists and will be overwritten"
end
LOGGER_TYPE_MAPPING[logger_type] = logger_constructor
return nothing
end
const LOGGER_TYPE_MAPPING = Dict(
"SeqLogger" => load_seqlogger,
"ConsoleLogger" => load_consolelogger,
"FileLogger" => load_filelogger,
"AdvancedFileLogger" => load_advanced_filelogger,
)
# ====================================================================
# Transformation Function and Register
# ====================================================================
"""
get_transformation_function(key::String)
Convert a string (from config) into a transformation function.
By default, the following transformation functions are supported:
- `"identity"` → [`identity`](@ref): no transformation
- `"add_timestamp"` → [`add_timestamp`](@ref): add timestamp at the beginning of log message
Use [`register_transformation_function!`](@ref) to add custom transformation functions.
"""
function get_transformation_function(key::String)
return LOGGER_TRANSFORMATION_MAPPING[key]
end
"""
register_transformation_function!(key::AbstractString, transformation_function::Function)
Register new transformation function.
Registering enables the user to use custom transformation functions, defined outside of `SeqLoggers`,
to be used with [`load_logger_from_config`](@ref).
"""
function register_transformation_function!(key::AbstractString, transformation_function::Function)
LOGGER_TRANSFORMATION_MAPPING[key] = transformation_function
return nothing
end
const STANDARD_DATETIME_FORMAT = "yyyy-mm-dd HH:MM:SS"
"""
add_timestamp(logger::AbstractLogger)
Logger transformation function that prepends a timestamp to a logging message.
"""
add_timestamp(logger) = TransformerLogger(logger) do log
merge(log, (; message="$(Dates.format(now(), STANDARD_DATETIME_FORMAT)): $(log.message)"))
end
const LOGGER_TRANSFORMATION_MAPPING = Dict(
"identity" => identity,
"add_timestamp" => add_timestamp,
)
# ====================================================================
# Log Level
# ====================================================================
"""
get_log_level(key::String)::Logging.LogLevel
Return the `Loggin.LogLevel` corresponding to the input string.
"""
function get_log_level(key::String)
log_level = get(LOG_LEVEL_MAPPING, uppercase(key), nothing)
if isnothing(key)
throw(
ArgumentError(
"There is no log level corresponding to the key $key." *
"Available options are $(collect(keys(LOG_LEVEL_MAPPING)))"
)
)
end
return log_level
end
const LOG_LEVEL_MAPPING = Dict(
"INFO" => Logging.Info,
"INFORMATOIN" => Logging.Info,
"DEBUG" => Logging.Debug,
"WARN" => Logging.Warn,
"WARNING" => Logging.Warn,
"ERROR" => Logging.Error,
)
| [
2,
38093,
18604,
198,
2,
8778,
20615,
5972,
1362,
198,
2,
38093,
18604,
198,
37811,
198,
220,
220,
220,
3440,
62,
6404,
1362,
62,
6738,
62,
11250,
7,
7753,
62,
6978,
3712,
23839,
10100,
2599,
25,
51,
1453,
11187,
1362,
198,
198,
16447,
257,
5929,
49706,
422,
257,
4566,
2393,
3108,
13,
198,
198,
21017,
5740,
198,
32,
5929,
49706,
318,
257,
4600,
51,
1453,
11187,
1362,
63,
2878,
326,
3578,
284,
3758,
262,
976,
2604,
3275,
284,
220,
198,
439,
2604,
5355,
3017,
287,
262,
5929,
49706,
379,
1752,
13,
198,
37811,
198,
8818,
3440,
62,
6404,
1362,
62,
6738,
62,
11250,
7,
7753,
62,
6978,
3712,
23839,
10100,
8,
198,
220,
220,
220,
33918,
62,
8841,
796,
1100,
7,
7753,
62,
6978,
11,
10903,
8,
198,
220,
220,
220,
33918,
18,
62,
11600,
796,
19449,
18,
13,
961,
7,
17752,
62,
8841,
8,
1303,
11,
360,
713,
198,
220,
220,
220,
1441,
3440,
62,
6404,
1362,
62,
6738,
62,
11250,
7,
17752,
18,
62,
11600,
8,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
3440,
62,
6404,
1362,
62,
6738,
62,
11250,
7,
11250,
3712,
35,
713,
2599,
25,
51,
1453,
11187,
1362,
198,
198,
16447,
257,
4600,
51,
1453,
11187,
263,
47671,
257,
4947,
286,
49706,
11,
422,
257,
8398,
4600,
35,
713,
44646,
198,
198,
21017,
5740,
198,
12,
220,
317,
4600,
51,
1453,
11187,
1362,
63,
2878,
3578,
284,
3758,
262,
976,
2604,
3275,
220,
284,
220,
477,
2604,
5355,
3017,
287,
262,
4600,
51,
1453,
11187,
263,
63,
379,
1752,
13,
198,
12,
383,
8398,
4600,
35,
713,
63,
4433,
355,
4600,
1,
6404,
2667,
1,
63,
2214,
11,
766,
1672,
329,
517,
3307,
13,
198,
198,
21017,
17934,
220,
198,
15506,
63,
73,
43640,
198,
11250,
796,
360,
713,
7,
198,
220,
220,
220,
2644,
11,
198,
220,
220,
220,
366,
6404,
2667,
1,
5218,
685,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
49706,
62,
4906,
5218,
49706,
62,
11250,
62,
11600,
11,
198,
220,
220,
220,
220,
220,
220,
220,
366,
4653,
80,
11187,
1362,
1,
5218,
360,
713,
7,
986,
828,
198,
220,
220,
220,
220,
220,
220,
220,
2644,
198,
220,
220,
220,
16589,
198,
220,
220,
220,
2644,
198,
8,
198,
15506,
63,
198,
198,
21017,
16409,
198,
63,
51,
1453,
11187,
1362,
63,
355,
5447,
287,
4600,
11250,
63,
198,
37811,
198,
8818,
3440,
62,
6404,
1362,
62,
6738,
62,
11250,
7,
11250,
3712,
23839,
35,
713,
8,
198,
220,
220,
220,
2604,
5355,
796,
685,
198,
220,
220,
220,
220,
220,
220,
220,
651,
62,
6404,
1362,
7,
8841,
7,
6404,
1362,
62,
4906,
828,
49706,
62,
4125,
6359,
8,
329,
357,
6404,
1362,
62,
4906,
11,
49706,
62,
4125,
6359,
8,
287,
4566,
14692,
6404,
2667,
8973,
198,
220,
220,
220,
2361,
198,
220,
220,
220,
1441,
49350,
11187,
1362,
7,
6404,
5355,
23029,
198,
437,
198,
198,
2,
38093,
18604,
198,
2,
8778,
14206,
5972,
1362,
198,
2,
38093,
18604,
198,
37811,
198,
220,
220,
220,
3440,
62,
41068,
6404,
1362,
7,
6404,
1362,
62,
11250,
3712,
23839,
35,
713,
2599,
25,
38176,
90,
4653,
80,
11187,
1362,
11,
3602,
16354,
11187,
1362,
92,
198,
198,
13615,
257,
4600,
4653,
80,
11187,
1362,
63,
393,
4600,
8291,
16354,
11187,
1362,
63,
1864,
284,
4600,
6404,
1362,
62,
11250,
44646,
198,
198,
21017,
17056,
40117,
198,
12,
4600,
1,
15388,
62,
6371,
1,
63,
1377,
2672,
198,
12,
4600,
1,
1084,
62,
5715,
1,
63,
1377,
2672,
357,
63,
1,
30531,
1600,
366,
10778,
1600,
366,
37771,
1600,
366,
24908,
1,
63,
8,
198,
12,
4600,
1,
7645,
1161,
1,
63,
1377,
11902,
11,
4277,
4600,
738,
414,
63,
198,
12,
4600,
1,
15042,
62,
2539,
1,
63,
1377,
11902,
11,
4277,
4600,
15931,
63,
198,
12,
4600,
1,
43501,
62,
7857,
1,
63,
1377,
11902,
11,
4277,
4600,
940,
63,
198,
198,
3237,
584,
4566,
10007,
389,
973,
355,
3298,
1785,
6608,
13,
198,
198,
21017,
17934,
198,
15506,
63,
73,
43640,
198,
6404,
62,
11600,
796,
360,
713,
7,
198,
220,
220,
220,
366,
15388,
62,
6371,
1,
5218,
366,
4023,
1378,
7266,
32656,
23349,
25,
20,
33660,
14,
1600,
198,
220,
220,
220,
366,
1084,
62,
5715,
1,
5218,
366,
10778,
1600,
198,
220,
220,
220,
366,
43501,
62,
7857,
1,
5218,
1105,
11,
198,
220,
220,
220,
366,
4677,
1,
5218,
366,
4653,
80,
11187,
5355,
62,
14402,
1600,
198,
220,
220,
220,
366,
4834,
85,
1,
5218,
366,
14402,
1,
198,
8,
198,
41068,
62,
6404,
1362,
796,
1001,
80,
11187,
5355,
13,
2220,
62,
41068,
6404,
1362,
7,
6404,
62,
11600,
8,
198,
15506,
63,
198,
37811,
198,
8818,
3440,
62,
41068,
6404,
1362,
7,
6404,
1362,
62,
11250,
3712,
23839,
35,
713,
8,
198,
220,
220,
220,
4382,
62,
6371,
796,
49706,
62,
11250,
14692,
15388,
62,
6371,
8973,
198,
220,
220,
220,
949,
62,
5715,
796,
49706,
62,
11250,
14692,
1084,
62,
5715,
8973,
930,
29,
651,
62,
6404,
62,
5715,
198,
220,
220,
220,
13389,
62,
2536,
796,
651,
7,
6404,
1362,
62,
11250,
11,
366,
7645,
1161,
1600,
366,
738,
414,
4943,
198,
220,
220,
220,
13389,
796,
13389,
62,
2536,
930,
29,
651,
62,
7645,
1161,
62,
8818,
628,
220,
220,
220,
1303,
13610,
257,
34441,
51,
29291,
422,
5637,
17056,
7383,
8367,
350,
3468,
198,
220,
220,
220,
479,
86,
853,
62,
13083,
796,
8106,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1994,
4613,
4731,
7,
2539,
8,
18872,
231,
14631,
15388,
62,
6371,
1600,
366,
1084,
62,
5715,
1600,
366,
7645,
1161,
33116,
8251,
7,
6404,
1362,
62,
11250,
8,
198,
220,
220,
220,
1267,
198,
220,
220,
220,
479,
86,
853,
62,
13083,
62,
14933,
796,
309,
29291,
7,
13940,
23650,
7,
2539,
8,
329,
1994,
287,
479,
86,
853,
62,
13083,
8,
198,
220,
220,
220,
479,
86,
853,
62,
13083,
62,
27160,
796,
685,
6404,
1362,
62,
11250,
58,
2539,
60,
329,
1994,
287,
479,
86,
853,
62,
13083,
60,
198,
220,
220,
220,
479,
86,
853,
62,
13083,
796,
34441,
51,
29291,
90,
46265,
853,
62,
13083,
62,
14933,
92,
7,
46265,
853,
62,
13083,
62,
27160,
8,
198,
220,
220,
220,
1441,
1001,
80,
11187,
1362,
7,
198,
220,
220,
220,
220,
220,
220,
220,
4382,
62,
6371,
26,
949,
62,
5715,
28,
1084,
62,
5715,
11,
479,
86,
853,
62,
13083,
986,
198,
220,
220,
220,
1267,
930,
29,
13389,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
3440,
62,
41947,
6404,
1362,
7,
6404,
1362,
62,
11250,
3712,
23839,
35,
713,
2599,
25,
38176,
90,
47581,
11187,
1362,
11,
3602,
16354,
11187,
1362,
92,
198,
198,
13615,
257,
4600,
47581,
11187,
1362,
63,
393,
4600,
8291,
16354,
11187,
1362,
63,
1864,
284,
4600,
6404,
1362,
62,
11250,
44646,
198,
198,
21017,
17056,
40117,
198,
12,
4600,
1,
1084,
62,
5715,
1,
63,
1377,
2672,
357,
63,
1,
30531,
1600,
366,
10778,
1600,
366,
37771,
1600,
366,
24908,
1,
63,
8,
198,
12,
4600,
1,
7645,
1161,
1,
63,
1377,
11902,
11,
4277,
4600,
738,
414,
63,
198,
198,
21017,
17934,
198,
15506,
63,
73,
43640,
198,
6404,
2667,
62,
11250,
796,
360,
713,
7,
198,
220,
220,
220,
366,
1084,
62,
5715,
1,
5218,
366,
24908,
1600,
198,
220,
220,
220,
366,
7645,
1161,
1,
5218,
366,
2860,
62,
16514,
27823,
1600,
198,
8,
198,
198,
41068,
62,
6404,
1362,
796,
1001,
80,
11187,
5355,
13,
2220,
62,
41947,
6404,
1362,
7,
6404,
62,
11600,
8,
198,
15506,
63,
198,
37811,
198,
8818,
3440,
62,
41947,
6404,
1362,
7,
6404,
1362,
62,
11250,
3712,
23839,
35,
713,
8,
198,
220,
220,
220,
949,
62,
5715,
796,
49706,
62,
11250,
14692,
1084,
62,
5715,
8973,
930,
29,
651,
62,
6404,
62,
5715,
198,
220,
220,
220,
13389,
62,
2536,
796,
651,
7,
6404,
1362,
62,
11250,
11,
366,
7645,
1161,
1600,
366,
738,
414,
4943,
198,
220,
220,
220,
13389,
796,
13389,
62,
2536,
930,
29,
651,
62,
7645,
1161,
62,
8818,
198,
220,
220,
220,
1441,
24371,
11187,
1362,
7,
301,
1082,
81,
11,
949,
62,
5715,
8,
930,
29,
13389,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
3440,
62,
41947,
6404,
1362,
7,
6404,
1362,
62,
11250,
3712,
23839,
35,
713,
2599,
25,
23839,
11187,
1362,
198,
198,
13615,
257,
4600,
9452,
4971,
11187,
1362,
90,
8979,
11187,
1362,
92,
63,
393,
4600,
8291,
16354,
11187,
1362,
63,
1864,
284,
4600,
6404,
1362,
62,
11250,
44646,
198,
198,
21017,
17056,
40117,
198,
12,
4600,
1,
7753,
62,
6978,
1,
63,
1377,
2672,
198,
12,
4600,
1,
1084,
62,
5715,
1,
63,
1377,
2672,
357,
63,
1,
30531,
1600,
366,
10778,
1600,
366,
37771,
1600,
366,
24908,
1,
63,
8,
198,
12,
4600,
1,
33295,
1,
63,
1377,
11902,
11,
4277,
4600,
7942,
47671,
24443,
284,
2393,
611,
4600,
7942,
47671,
4306,
40122,
378,
2393,
13,
357,
6214,
685,
63,
11187,
2667,
11627,
8847,
13,
8979,
11187,
1362,
63,
16151,
31,
5420,
8,
329,
517,
1321,
2014,
198,
12,
4600,
1,
7645,
1161,
1,
63,
1377,
11902,
11,
4277,
4600,
738,
414,
63,
628,
198,
21017,
17934,
198,
15506,
63,
73,
43640,
198,
6404,
2667,
62,
11250,
796,
360,
713,
7,
198,
220,
220,
220,
366,
7753,
62,
6978,
1,
5218,
366,
34,
14079,
30782,
14,
9288,
13,
6404,
1600,
198,
220,
220,
220,
366,
1084,
62,
5715,
1,
5218,
366,
24908,
1600,
198,
220,
220,
220,
366,
33295,
1,
5218,
2081,
11,
198,
220,
220,
220,
366,
7645,
1161,
1,
5218,
366,
2860,
62,
16514,
27823,
1600,
198,
8,
198,
41068,
62,
6404,
1362,
796,
1001,
80,
11187,
5355,
13,
2220,
62,
7753,
6404,
1362,
7,
6404,
62,
11600,
8,
198,
15506,
63,
198,
37811,
198,
8818,
3440,
62,
7753,
6404,
1362,
7,
6404,
1362,
62,
11250,
3712,
23839,
35,
713,
8,
198,
220,
220,
220,
949,
62,
5715,
796,
49706,
62,
11250,
14692,
1084,
62,
5715,
8973,
930,
29,
651,
62,
6404,
62,
5715,
198,
220,
220,
220,
2393,
62,
6978,
796,
49706,
62,
11250,
14692,
7753,
62,
6978,
8973,
198,
220,
220,
220,
24443,
796,
651,
7,
6404,
1362,
62,
11250,
11,
366,
33295,
1600,
2081,
8,
198,
220,
220,
220,
13389,
62,
2536,
796,
651,
7,
6404,
1362,
62,
11250,
11,
366,
7645,
1161,
1600,
366,
738,
414,
4943,
198,
220,
220,
220,
13389,
796,
13389,
62,
2536,
930,
29,
651,
62,
7645,
1161,
62,
8818,
198,
220,
220,
220,
1441,
1855,
4971,
11187,
1362,
7,
8979,
11187,
1362,
7,
7753,
62,
6978,
26,
24443,
28,
33295,
828,
949,
62,
5715,
8,
930,
29,
13389,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
3440,
62,
32225,
2903,
62,
7753,
6404,
1362,
7,
6404,
1362,
62,
11250,
3712,
23839,
35,
713,
2599,
25,
23839,
11187,
1362,
198,
198,
13615,
257,
4600,
27354,
8079,
24864,
803,
8979,
11187,
1362,
63,
393,
4600,
8291,
16354,
11187,
1362,
63,
1864,
284,
4600,
6404,
1362,
62,
11250,
44646,
198,
198,
21017,
17056,
40117,
198,
12,
4600,
1,
15908,
62,
6978,
1,
63,
1377,
2672,
198,
12,
4600,
1,
1084,
62,
5715,
1,
63,
1377,
2672,
357,
63,
1,
30531,
1600,
366,
10778,
1600,
366,
37771,
1600,
366,
24908,
1,
63,
8,
198,
12,
4600,
1,
7753,
62,
3672,
62,
33279,
1,
63,
1377,
2672,
304,
13,
70,
13,
4600,
1,
6852,
64,
6852,
66,
6852,
66,
6852,
68,
6852,
82,
6852,
82,
12,
26314,
26314,
12,
3020,
12,
1860,
12,
16768,
12,
12038,
13,
6852,
75,
6852,
78,
6852,
70,
1,
63,
198,
12,
4600,
1,
7645,
1161,
1,
63,
1377,
11902,
11,
4277,
4600,
738,
414,
63,
198,
198,
21017,
17934,
198,
15506,
63,
73,
43640,
198,
6404,
2667,
62,
11250,
796,
360,
713,
7,
198,
220,
220,
220,
366,
15908,
62,
6978,
1,
5218,
366,
34,
14079,
30782,
1600,
198,
220,
220,
220,
366,
1084,
62,
5715,
1,
5218,
366,
24908,
1600,
198,
220,
220,
220,
366,
7753,
62,
3672,
62,
33279,
1,
5218,
366,
6852,
64,
6852,
66,
6852,
66,
6852,
68,
6852,
82,
6852,
82,
12,
26314,
26314,
12,
3020,
12,
1860,
12,
16768,
12,
12038,
13,
6852,
75,
6852,
78,
6852,
70,
1600,
198,
220,
220,
220,
366,
7645,
1161,
1,
5218,
366,
2860,
62,
16514,
27823,
1600,
198,
8,
198,
41068,
62,
6404,
1362,
796,
1001,
80,
11187,
5355,
13,
2220,
62,
32225,
2903,
62,
7753,
6404,
1362,
7,
6404,
62,
11600,
8,
198,
15506,
63,
198,
37811,
198,
8818,
3440,
62,
32225,
2903,
62,
7753,
6404,
1362,
7,
6404,
1362,
62,
11250,
3712,
23839,
35,
713,
8,
198,
220,
220,
220,
949,
62,
5715,
796,
49706,
62,
11250,
14692,
1084,
62,
5715,
8973,
930,
29,
651,
62,
6404,
62,
5715,
198,
220,
220,
220,
26672,
62,
6978,
796,
49706,
62,
11250,
14692,
15908,
62,
6978,
8973,
198,
220,
220,
220,
2393,
62,
3672,
62,
33279,
796,
49706,
62,
11250,
14692,
7753,
62,
3672,
62,
33279,
8973,
628,
220,
220,
220,
13389,
62,
2536,
796,
651,
7,
6404,
1362,
62,
11250,
11,
366,
7645,
1161,
1600,
366,
738,
414,
4943,
198,
220,
220,
220,
13389,
796,
13389,
62,
2536,
930,
29,
651,
62,
7645,
1161,
62,
8818,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1441,
13435,
8979,
11187,
1362,
7,
198,
220,
220,
220,
220,
220,
220,
220,
26672,
62,
6978,
11,
220,
198,
220,
220,
220,
220,
220,
220,
220,
2393,
62,
3672,
62,
33279,
26,
220,
198,
220,
220,
220,
220,
220,
220,
220,
2604,
62,
18982,
62,
8818,
28,
4798,
62,
20307,
62,
18982,
11,
220,
198,
220,
220,
220,
220,
220,
220,
220,
949,
62,
5715,
28,
1084,
62,
5715,
198,
220,
220,
220,
1267,
930,
29,
13389,
198,
437,
198,
198,
2,
38093,
18604,
198,
2,
5972,
1362,
5994,
337,
5912,
290,
17296,
198,
2,
38093,
18604,
198,
37811,
198,
220,
220,
220,
651,
62,
6404,
1362,
7,
6404,
1362,
62,
4906,
3712,
23839,
10100,
11,
49706,
62,
11250,
3712,
23839,
35,
713,
2599,
25,
23839,
11187,
1362,
198,
198,
16447,
49706,
2878,
422,
49706,
2099,
1438,
290,
4600,
35,
713,
63,
351,
2672,
10007,
13,
198,
198,
3886,
4277,
11,
262,
1708,
49706,
3858,
389,
4855,
25,
198,
12,
4600,
1,
4653,
80,
11187,
1362,
1,
63,
15168,
685,
63,
4653,
80,
11187,
1362,
63,
16151,
31,
5420,
8,
198,
12,
4600,
1,
47581,
11187,
1362,
1,
63,
15168,
685,
63,
47581,
11187,
1362,
63,
16151,
31,
5420,
8,
198,
12,
4600,
1,
8979,
11187,
1362,
1,
63,
15168,
685,
63,
8979,
11187,
1362,
63,
16151,
31,
5420,
8,
198,
198,
11041,
685,
63,
30238,
62,
6404,
1362,
0,
63,
16151,
31,
5420,
8,
284,
751,
2183,
4600,
23839,
11187,
1362,
63,
82,
13,
198,
37811,
198,
8818,
651,
62,
6404,
1362,
7,
6404,
1362,
62,
4906,
3712,
23839,
10100,
11,
49706,
62,
11250,
3712,
23839,
35,
713,
8,
198,
220,
220,
220,
49706,
62,
41571,
273,
796,
651,
7,
25294,
30373,
62,
25216,
62,
44,
24805,
2751,
11,
49706,
62,
4906,
11,
2147,
8,
198,
220,
220,
220,
611,
318,
22366,
7,
6404,
1362,
62,
41571,
273,
8,
198,
220,
220,
220,
220,
220,
220,
220,
3714,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
45751,
12331,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
1858,
318,
645,
49706,
11188,
284,
262,
1994,
4600,
3,
6404,
1362,
62,
4906,
44646,
366,
1635,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
10493,
3689,
389,
29568,
33327,
7,
13083,
7,
25294,
30373,
62,
25216,
62,
44,
24805,
2751,
4008,
737,
366,
1635,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
11041,
4600,
30238,
62,
6404,
1362,
0,
63,
284,
751,
649,
49706,
3858,
526,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1267,
198,
220,
220,
220,
220,
220,
220,
220,
1267,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
49706,
62,
41571,
273,
7,
6404,
1362,
62,
11250,
8,
198,
437,
198,
198,
37811,
220,
198,
220,
220,
220,
7881,
62,
6404,
1362,
0,
7,
6404,
1362,
62,
4906,
3712,
23839,
10100,
11,
49706,
62,
41571,
273,
3712,
22203,
8,
198,
198,
38804,
257,
649,
49706,
2099,
13,
198,
198,
38804,
278,
13536,
262,
2836,
284,
779,
2183,
4600,
23839,
11187,
1362,
63,
2878,
11,
5447,
2354,
286,
4600,
4653,
80,
11187,
5355,
47671,
198,
1462,
307,
973,
351,
685,
63,
2220,
62,
6404,
1362,
62,
6738,
62,
11250,
63,
16151,
31,
5420,
737,
198,
37811,
198,
8818,
7881,
62,
6404,
1362,
0,
7,
6404,
1362,
62,
4906,
3712,
23839,
10100,
11,
49706,
62,
41571,
273,
3712,
22203,
8,
198,
220,
220,
220,
611,
468,
2539,
7,
25294,
30373,
62,
25216,
62,
44,
24805,
2751,
11,
49706,
62,
4906,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
40539,
366,
11187,
1362,
2099,
4600,
3,
6404,
1362,
62,
4906,
63,
1541,
7160,
290,
481,
307,
6993,
9108,
1,
198,
220,
220,
220,
886,
198,
220,
220,
220,
41605,
30373,
62,
25216,
62,
44,
24805,
2751,
58,
6404,
1362,
62,
4906,
60,
796,
49706,
62,
41571,
273,
198,
220,
220,
220,
1441,
2147,
198,
437,
198,
198,
9979,
41605,
30373,
62,
25216,
62,
44,
24805,
2751,
796,
360,
713,
7,
198,
220,
220,
220,
366,
4653,
80,
11187,
1362,
1,
5218,
3440,
62,
41068,
6404,
1362,
11,
198,
220,
220,
220,
366,
47581,
11187,
1362,
1,
5218,
3440,
62,
41947,
6404,
1362,
11,
198,
220,
220,
220,
366,
8979,
11187,
1362,
1,
5218,
3440,
62,
7753,
6404,
1362,
11,
198,
220,
220,
220,
366,
28809,
8979,
11187,
1362,
1,
5218,
3440,
62,
32225,
2903,
62,
7753,
6404,
1362,
11,
198,
8,
198,
198,
2,
38093,
18604,
198,
2,
49127,
15553,
290,
17296,
198,
2,
38093,
18604,
198,
198,
37811,
198,
220,
220,
220,
651,
62,
7645,
1161,
62,
8818,
7,
2539,
3712,
10100,
8,
198,
198,
3103,
1851,
257,
4731,
357,
6738,
4566,
8,
656,
257,
13389,
2163,
13,
198,
198,
3886,
4277,
11,
262,
1708,
13389,
5499,
389,
4855,
25,
198,
12,
4600,
1,
738,
414,
1,
63,
15168,
685,
63,
738,
414,
63,
16151,
31,
5420,
2599,
645,
13389,
198,
12,
4600,
1,
2860,
62,
16514,
27823,
1,
63,
15168,
685,
63,
2860,
62,
16514,
27823,
63,
16151,
31,
5420,
2599,
751,
41033,
379,
262,
3726,
286,
2604,
3275,
198,
198,
11041,
685,
63,
30238,
62,
7645,
1161,
62,
8818,
0,
63,
16151,
31,
5420,
8,
284,
751,
2183,
13389,
5499,
13,
198,
37811,
198,
8818,
651,
62,
7645,
1161,
62,
8818,
7,
2539,
3712,
10100,
8,
198,
220,
220,
220,
1441,
41605,
30373,
62,
5446,
15037,
35036,
62,
44,
24805,
2751,
58,
2539,
60,
198,
437,
198,
198,
37811,
198,
220,
220,
220,
7881,
62,
7645,
1161,
62,
8818,
0,
7,
2539,
3712,
23839,
10100,
11,
13389,
62,
8818,
3712,
22203,
8,
198,
198,
38804,
649,
13389,
2163,
13,
198,
198,
38804,
278,
13536,
262,
2836,
284,
779,
2183,
13389,
5499,
11,
5447,
2354,
286,
4600,
4653,
80,
11187,
5355,
47671,
198,
1462,
307,
973,
351,
685,
63,
2220,
62,
6404,
1362,
62,
6738,
62,
11250,
63,
16151,
31,
5420,
737,
198,
37811,
198,
8818,
7881,
62,
7645,
1161,
62,
8818,
0,
7,
2539,
3712,
23839,
10100,
11,
13389,
62,
8818,
3712,
22203,
8,
198,
220,
220,
220,
41605,
30373,
62,
5446,
15037,
35036,
62,
44,
24805,
2751,
58,
2539,
60,
796,
13389,
62,
8818,
198,
220,
220,
220,
1441,
2147,
198,
437,
198,
198,
9979,
49053,
9795,
62,
35,
1404,
2767,
12789,
62,
21389,
1404,
796,
366,
22556,
22556,
12,
3020,
12,
1860,
47138,
25,
12038,
25,
5432,
1,
198,
198,
37811,
198,
2860,
62,
16514,
27823,
7,
6404,
1362,
3712,
23839,
11187,
1362,
8,
198,
198,
11187,
1362,
13389,
2163,
326,
3143,
2412,
257,
41033,
284,
257,
18931,
3275,
13,
198,
37811,
198,
2860,
62,
16514,
27823,
7,
6404,
1362,
8,
796,
3602,
16354,
11187,
1362,
7,
6404,
1362,
8,
466,
2604,
198,
220,
220,
220,
20121,
7,
6404,
11,
357,
26,
3275,
2625,
3,
7,
35,
689,
13,
18982,
7,
2197,
22784,
49053,
9795,
62,
35,
1404,
2767,
12789,
62,
21389,
1404,
8,
2599,
29568,
6404,
13,
20500,
16725,
4008,
198,
437,
198,
198,
9979,
41605,
30373,
62,
5446,
15037,
35036,
62,
44,
24805,
2751,
796,
360,
713,
7,
198,
220,
220,
220,
366,
738,
414,
1,
5218,
5369,
11,
198,
220,
220,
220,
366,
2860,
62,
16514,
27823,
1,
5218,
751,
62,
16514,
27823,
11,
198,
8,
628,
198,
2,
38093,
18604,
198,
2,
5972,
5684,
198,
2,
38093,
18604,
198,
37811,
198,
220,
220,
220,
651,
62,
6404,
62,
5715,
7,
2539,
3712,
10100,
2599,
25,
11187,
2667,
13,
11187,
4971,
198,
198,
13615,
262,
4600,
11187,
1655,
13,
11187,
4971,
63,
11188,
284,
262,
5128,
4731,
13,
198,
37811,
198,
8818,
651,
62,
6404,
62,
5715,
7,
2539,
3712,
10100,
8,
198,
220,
220,
220,
2604,
62,
5715,
796,
651,
7,
25294,
62,
2538,
18697,
62,
44,
24805,
2751,
11,
334,
39921,
589,
7,
2539,
828,
2147,
8,
198,
220,
220,
220,
611,
318,
22366,
7,
2539,
8,
198,
220,
220,
220,
220,
220,
220,
220,
3714,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
45751,
12331,
7,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
1858,
318,
645,
2604,
1241,
11188,
284,
262,
1994,
720,
2539,
526,
1635,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
366,
10493,
3689,
389,
29568,
33327,
7,
13083,
7,
25294,
62,
2538,
18697,
62,
44,
24805,
2751,
4008,
16725,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1267,
198,
220,
220,
220,
220,
220,
220,
220,
1267,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
2604,
62,
5715,
198,
437,
198,
198,
9979,
41605,
62,
2538,
18697,
62,
44,
24805,
2751,
796,
360,
713,
7,
198,
220,
220,
220,
366,
10778,
1,
5218,
5972,
2667,
13,
12360,
11,
198,
220,
220,
220,
366,
1268,
21389,
1404,
46,
1268,
1,
5218,
5972,
2667,
13,
12360,
11,
198,
220,
220,
220,
366,
30531,
1,
5218,
5972,
2667,
13,
27509,
11,
198,
220,
220,
220,
366,
37771,
1,
5218,
5972,
2667,
13,
54,
1501,
11,
198,
220,
220,
220,
366,
31502,
1,
5218,
5972,
2667,
13,
54,
1501,
11,
198,
220,
220,
220,
366,
24908,
1,
5218,
5972,
2667,
13,
12331,
11,
198,
8,
628
] | 2.877757 | 3,763 |
#=
A port of my Perl/Java/Python MakeRegex in Julia.
make_regex(words)
generates a regex for words. It use a simple approach which
combined common prefixes in generating the regex.
Some examples:
* words = ["a", "al", "all", "alla", "an", "ann", "anna", "annas", "ananas"]
regex: a(l(la?)?|n(anas|n(as?)?)?)?
* words: ["and", "at", "do", "end", "for", "in", "is", "not", "of", "or", "use"]
regex: (a(nd|t)|do|end|for|i[ns]|not|o[fr]|use)
There is a simple way of handling character classes
* words: ["price1", "price2", "price3", "price4"]
regex: price[1234]
If there is no common prefix then it just put '|' between the words
* words: ["this", "is", "a", "very", "boring", "example", "with", "no", "common", "prefix"]
regex: (a|boring|common|example|is|no|prefix|this|very|with)
Also, see the (very old) page for my Perl package MakeRegex: http://hakank.org/makeregex/index.html
The REAME file in that package states:
"""
The Perl package MakeRegex composes a regex-expression from a list of
words. It had been inspired by the emacs elisp module make-regex.el,
by Simon Marshall.
"""
This Julia program was created by Hakan Kjellerstrand, hakank@gmail.com
See also my Julia page: http://www.hakank.org/julia/
=#
#
# common_prefix(p, list)
#
# Here is where the main work is done. It's somewhat magically ported from my
# Perl/Java/Python versions...
#
function common_prefix(p, list)
list_len = length(list)
if list_len == 0
return ""
end
if list_len == 1
return p * join(list,"")
end
#
# fix for some - as of now - unknown bug. To fix in later version!
#
if p == "" && list[1] == "" && list[2] == ""
return ""
end
#
# * Collect all the strings with the same prefix-char
#
hash = Dict()
for word in sort(list)
prefix = suffixed_word = ""
if length(word) > 0
prefix, suffixed_word... = word
end
# put the suffix in the list of other suffixes for
# the this prefix
hash[prefix] = push!(get(hash,prefix,[]),suffixed_word)
end
#
# And recurse this list
#
all = []
for key in keys(hash)
comm = ""
values = hash[key]
if length(key) > 0
sort!(values)
comm = common_prefix(key, values)
end
# hack to be able to use the '?' char . Should be re-written!
if comm == ""
comm = " "
end
push!(all,comm)
end
sort!(all)
# paren: what to put in parenthesis ('()' or '[]') if anything
paren = ""
all_len = length(all)
if all_len == 1
paren = join(all,"")
else
len = maximum(length.(all))
joinChar = len != 1 ? '|' : ""
# joins all entries except for " "
join_str = mark = ""
count = 0
for w in all
got_hack_mark = w == " " ? true : false # This is a hack for handling '?'
if length(w) > 0 && w != " "
join_str *= w
if count < all_len-1
join_str *= joinChar
end
end
if got_hack_mark
mark = '?'
end
count = count + 1
end
paren = ""
if length(join_str) === 1
paren = join_str * mark
else
if len == 1
paren = '[' * join_str * ']' * mark
else
paren = '(' * join_str * ')' * mark
end
end
end
return p * paren
end
function make_regex(words)
replace.(words,r"([*?+])"=>s"\\\1") # replace meta characters
# We sort the words to induce more common prefixes
return common_prefix("", sort(words))
end
#
# check_regex(regex, words)
#
# Checks the regex againts a list of words.
#
function check_regex(regex, words)
p = Regex(regex)
for word in words
println(word, " matches", !occursin(p,word) ? " NOT!" : "")
end
end
tests = [
["all","alla"],
# A lot of Swedish words
[ "all", "alla", "alle", "alls", "palle", "palla", "pelle", "perkele",
"ann", "anna", "annas", "anders", "håkan", "ångest", "ärlig",
"solsken", "sture", "stina", "hörapparat", "hörsel", "hårig"],
["alla", "palla", "balla", "kalla", "all", "pall", "ball", "kall"],
# "ananas" is the Swedish word for pineapple
["a", "al", "all", "alla", "an", "ann", "anna", "annas", "ananas"],
["a", "an", "ann", "anna", "annan", "annas", "annans", "ananas", "ananasens"],
["a", "ab", "abc", "abcd", "abcde", "abcdef", "b", "bc", "bcd", "bcde", "bcdef",
"bcdefg", "abb", "abbc", "abbcc", "abbccdd"],
["this", "is", "a", "very", "boring", "example", "with", "no", "common", "prefix"],
["price1","price2","price3","price4"],
# This is from Marshall's make-regex.el
["and", "at", "do", "end", "for", "in", "is", "not", "of", "or", "use"],
# This is from Marshall's make-regex.el
["cond", "if", "while", "let*?", "prog1", "prog2", "progn",
"catch", "throw", "save-restriction", "save-excursion",
"save-window-excursion", "save-match-data", "unwind-protect",
"condition-case", "track-mouse"],
# This is from Marshall's make-regex.el
["abort", "abs", "accept", "access", "array",
"begin", "body", "case", "constant", "declare",
"delay", "delta", "digits", "else", "elsif", "entry",
"exception", "exit", "function", "generic", "goto",
"if", "others", "limited", "loop", "mod", "new",
"null", "out", "subtype", "package", "pragma",
"private", "procedure", "raise", "range", "record",
"rem", "renames", "return", "reverse", "select",
"separate", "task", "terminate", "then", "type",
"when", "while", "with", "xor"]
]
for t in tests
println("testing $t")
println(make_regex(t))
println()
end
#=
words = last(tests)
rx = make_regex(words)
println(words)
println("regex:$rx")
check_regex(rx,words)
=# | [
2,
28,
198,
220,
220,
220,
317,
2493,
286,
616,
24316,
14,
29584,
14,
37906,
6889,
3041,
25636,
287,
22300,
13,
628,
220,
220,
220,
787,
62,
260,
25636,
7,
10879,
8,
198,
220,
220,
220,
18616,
257,
40364,
329,
2456,
13,
632,
779,
257,
2829,
3164,
543,
220,
198,
220,
220,
220,
5929,
2219,
21231,
274,
287,
15453,
262,
40364,
13,
220,
628,
220,
220,
220,
2773,
6096,
25,
198,
220,
220,
220,
1635,
2456,
796,
14631,
64,
1600,
366,
282,
1600,
366,
439,
1600,
366,
30315,
1600,
366,
272,
1600,
366,
1236,
1600,
366,
7697,
1600,
366,
1236,
292,
1600,
366,
272,
15991,
8973,
198,
220,
220,
220,
220,
220,
40364,
25,
257,
7,
75,
7,
5031,
10091,
30,
91,
77,
7,
15991,
91,
77,
7,
292,
10091,
10091,
10091,
30,
628,
220,
220,
220,
1635,
2456,
25,
14631,
392,
1600,
366,
265,
1600,
366,
4598,
1600,
366,
437,
1600,
366,
1640,
1600,
366,
259,
1600,
366,
271,
1600,
366,
1662,
1600,
366,
1659,
1600,
366,
273,
1600,
366,
1904,
8973,
198,
220,
220,
220,
220,
220,
40364,
25,
357,
64,
7,
358,
91,
83,
14726,
4598,
91,
437,
91,
1640,
91,
72,
58,
5907,
60,
91,
1662,
91,
78,
58,
8310,
60,
91,
1904,
8,
628,
220,
220,
220,
1318,
318,
257,
2829,
835,
286,
9041,
2095,
6097,
198,
220,
220,
220,
1635,
2456,
25,
14631,
20888,
16,
1600,
366,
20888,
17,
1600,
366,
20888,
18,
1600,
366,
20888,
19,
8973,
198,
220,
220,
220,
220,
220,
40364,
25,
2756,
58,
1065,
2682,
60,
628,
198,
220,
220,
220,
1002,
612,
318,
645,
2219,
21231,
788,
340,
655,
1234,
705,
91,
6,
1022,
262,
2456,
220,
198,
220,
220,
220,
1635,
2456,
25,
14631,
5661,
1600,
366,
271,
1600,
366,
64,
1600,
366,
548,
1600,
366,
2865,
278,
1600,
366,
20688,
1600,
366,
4480,
1600,
366,
3919,
1600,
366,
11321,
1600,
366,
40290,
8973,
198,
220,
220,
220,
220,
220,
40364,
25,
357,
64,
91,
2865,
278,
91,
11321,
91,
20688,
91,
271,
91,
3919,
91,
40290,
91,
5661,
91,
548,
91,
4480,
8,
628,
198,
220,
220,
220,
4418,
11,
766,
262,
357,
548,
1468,
8,
2443,
329,
616,
24316,
5301,
6889,
3041,
25636,
25,
2638,
1378,
43573,
962,
13,
2398,
14,
15883,
260,
25636,
14,
9630,
13,
6494,
198,
220,
220,
220,
383,
4526,
10067,
2393,
287,
326,
5301,
2585,
25,
198,
220,
220,
220,
37227,
198,
220,
220,
220,
383,
24316,
5301,
6889,
3041,
25636,
552,
4629,
257,
40364,
12,
38011,
422,
257,
1351,
286,
198,
220,
220,
220,
2456,
13,
632,
550,
587,
7867,
416,
262,
795,
16436,
1288,
8802,
8265,
787,
12,
260,
25636,
13,
417,
11,
198,
220,
220,
220,
416,
11288,
13606,
13,
198,
220,
220,
220,
37227,
198,
220,
220,
220,
220,
198,
220,
220,
220,
770,
22300,
1430,
373,
2727,
416,
24734,
272,
509,
73,
12368,
2536,
392,
11,
387,
74,
962,
31,
14816,
13,
785,
198,
220,
220,
220,
4091,
635,
616,
22300,
2443,
25,
2638,
1378,
2503,
13,
43573,
962,
13,
2398,
14,
73,
43640,
14,
198,
198,
46249,
198,
198,
2,
220,
198,
2,
2219,
62,
40290,
7,
79,
11,
220,
1351,
8,
198,
2,
220,
198,
2,
3423,
318,
810,
262,
1388,
670,
318,
1760,
13,
632,
338,
6454,
34850,
49702,
422,
616,
198,
2,
24316,
14,
29584,
14,
37906,
6300,
986,
198,
2,
220,
198,
8818,
2219,
62,
40290,
7,
79,
11,
1351,
8,
220,
198,
220,
220,
220,
1351,
62,
11925,
796,
4129,
7,
4868,
8,
198,
220,
220,
220,
611,
1351,
62,
11925,
6624,
657,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
13538,
220,
198,
220,
220,
220,
886,
198,
220,
220,
220,
611,
1351,
62,
11925,
6624,
352,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
279,
1635,
4654,
7,
4868,
553,
4943,
198,
220,
220,
220,
886,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
4259,
329,
617,
532,
355,
286,
783,
532,
6439,
5434,
13,
1675,
4259,
287,
1568,
2196,
0,
198,
220,
220,
220,
1303,
220,
198,
220,
220,
220,
611,
279,
6624,
13538,
11405,
1351,
58,
16,
60,
6624,
13538,
11405,
1351,
58,
17,
60,
6624,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
13538,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
198,
220,
220,
220,
1303,
1635,
9745,
477,
262,
13042,
351,
262,
976,
21231,
12,
10641,
198,
220,
220,
220,
1303,
198,
220,
220,
220,
12234,
796,
360,
713,
3419,
198,
220,
220,
220,
329,
1573,
287,
3297,
7,
4868,
8,
198,
220,
220,
220,
220,
220,
220,
220,
21231,
796,
3027,
2966,
62,
4775,
796,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
611,
4129,
7,
4775,
8,
1875,
657,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
21231,
11,
3027,
2966,
62,
4775,
986,
796,
1573,
220,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
1234,
262,
35488,
287,
262,
1351,
286,
584,
35488,
274,
329,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
262,
428,
21231,
198,
220,
220,
220,
220,
220,
220,
220,
12234,
58,
40290,
60,
796,
4574,
0,
7,
1136,
7,
17831,
11,
40290,
17414,
46570,
37333,
2966,
62,
4775,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
220,
198,
220,
220,
220,
1303,
843,
664,
12321,
428,
1351,
198,
220,
220,
220,
1303,
220,
198,
220,
220,
220,
477,
796,
17635,
198,
220,
220,
220,
329,
1994,
287,
8251,
7,
17831,
8,
198,
220,
220,
220,
220,
220,
220,
220,
725,
796,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
3815,
796,
12234,
58,
2539,
60,
198,
220,
220,
220,
220,
220,
220,
220,
611,
4129,
7,
2539,
8,
1875,
657,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
3297,
0,
7,
27160,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
725,
796,
2219,
62,
40290,
7,
2539,
11,
3815,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
8156,
284,
307,
1498,
284,
779,
262,
705,
8348,
1149,
764,
10358,
307,
302,
12,
15266,
0,
198,
220,
220,
220,
220,
220,
220,
220,
611,
725,
6624,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
725,
796,
366,
366,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
439,
11,
9503,
8,
198,
220,
220,
220,
886,
220,
198,
220,
220,
220,
3297,
0,
7,
439,
8,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1303,
279,
5757,
25,
644,
284,
1234,
287,
2560,
8497,
19203,
3419,
6,
393,
705,
21737,
11537,
611,
1997,
198,
220,
220,
220,
279,
5757,
796,
13538,
198,
220,
220,
220,
477,
62,
11925,
796,
4129,
7,
439,
8,
198,
220,
220,
220,
611,
220,
477,
62,
11925,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
279,
5757,
796,
4654,
7,
439,
553,
4943,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
18896,
796,
5415,
7,
13664,
12195,
439,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
4654,
12441,
796,
18896,
14512,
352,
5633,
705,
91,
6,
1058,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
15449,
477,
12784,
2845,
329,
366,
366,
198,
220,
220,
220,
220,
220,
220,
220,
4654,
62,
2536,
796,
1317,
796,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
954,
796,
657,
198,
220,
220,
220,
220,
220,
220,
220,
329,
266,
287,
477,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1392,
62,
31153,
62,
4102,
796,
266,
6624,
366,
366,
5633,
2081,
1058,
3991,
1303,
770,
318,
257,
8156,
329,
9041,
705,
8348,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
4129,
7,
86,
8,
1875,
657,
11405,
266,
14512,
366,
366,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4654,
62,
2536,
1635,
28,
266,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
954,
1279,
477,
62,
11925,
12,
16,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4654,
62,
2536,
1635,
28,
220,
4654,
12441,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
628,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
1392,
62,
31153,
62,
4102,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1317,
796,
705,
8348,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
954,
796,
954,
1343,
352,
198,
220,
220,
220,
220,
220,
220,
220,
886,
628,
220,
220,
220,
220,
220,
220,
220,
279,
5757,
796,
13538,
198,
220,
220,
220,
220,
220,
220,
220,
611,
4129,
7,
22179,
62,
2536,
8,
24844,
352,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
279,
5757,
796,
4654,
62,
2536,
1635,
1317,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
18896,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
279,
5757,
796,
705,
17816,
1635,
4654,
62,
2536,
1635,
705,
49946,
1635,
1317,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
279,
5757,
796,
705,
10786,
1635,
4654,
62,
2536,
1635,
705,
33047,
1635,
1317,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
220,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
279,
1635,
279,
5757,
198,
198,
437,
198,
198,
8818,
787,
62,
260,
25636,
7,
10879,
8,
220,
198,
220,
220,
220,
6330,
12195,
10879,
11,
81,
18109,
58,
9,
30,
10,
12962,
1,
14804,
82,
1,
6852,
59,
16,
4943,
1303,
6330,
13634,
3435,
198,
220,
220,
220,
1303,
775,
3297,
262,
2456,
284,
21155,
517,
2219,
21231,
274,
198,
220,
220,
220,
1441,
2219,
62,
40290,
7203,
1600,
3297,
7,
10879,
4008,
198,
198,
437,
198,
198,
2,
198,
2,
2198,
62,
260,
25636,
7,
260,
25636,
11,
2456,
8,
198,
2,
220,
198,
2,
47719,
262,
40364,
757,
912,
257,
1351,
286,
2456,
13,
198,
2,
220,
198,
8818,
2198,
62,
260,
25636,
7,
260,
25636,
11,
2456,
8,
198,
220,
220,
220,
279,
796,
797,
25636,
7,
260,
25636,
8,
198,
220,
220,
220,
329,
1573,
287,
2456,
198,
220,
220,
220,
220,
220,
220,
220,
44872,
7,
4775,
11,
366,
7466,
1600,
5145,
13966,
1834,
259,
7,
79,
11,
4775,
8,
5633,
366,
5626,
2474,
1058,
366,
4943,
198,
220,
220,
220,
886,
220,
198,
437,
628,
198,
41989,
796,
685,
198,
220,
220,
220,
14631,
439,
2430,
30315,
33116,
628,
220,
220,
220,
1303,
317,
1256,
286,
14023,
2456,
198,
220,
220,
220,
685,
366,
439,
1600,
366,
30315,
1600,
366,
6765,
1600,
366,
5691,
1600,
366,
79,
6765,
1600,
366,
79,
30315,
1600,
366,
79,
13485,
1600,
366,
525,
365,
293,
1600,
198,
220,
220,
220,
220,
220,
366,
1236,
1600,
366,
7697,
1600,
366,
1236,
292,
1600,
366,
45070,
1600,
366,
71,
29090,
27541,
1600,
366,
29090,
782,
395,
1600,
366,
11033,
81,
4604,
1600,
220,
198,
220,
220,
220,
220,
220,
366,
82,
10220,
3464,
1600,
366,
301,
495,
1600,
366,
301,
1437,
1600,
366,
71,
9101,
430,
381,
34174,
1600,
366,
71,
30570,
741,
1600,
366,
71,
29090,
4359,
33116,
628,
220,
220,
220,
14631,
30315,
1600,
366,
79,
30315,
1600,
366,
1894,
64,
1600,
366,
74,
30315,
1600,
366,
439,
1600,
366,
79,
439,
1600,
366,
1894,
1600,
366,
74,
439,
33116,
628,
220,
220,
220,
1303,
366,
272,
15991,
1,
318,
262,
14023,
1573,
329,
45540,
198,
220,
220,
220,
14631,
64,
1600,
366,
282,
1600,
366,
439,
1600,
366,
30315,
1600,
366,
272,
1600,
366,
1236,
1600,
366,
7697,
1600,
366,
1236,
292,
1600,
366,
272,
15991,
33116,
628,
220,
220,
220,
14631,
64,
1600,
366,
272,
1600,
366,
1236,
1600,
366,
7697,
1600,
366,
1236,
272,
1600,
366,
1236,
292,
1600,
366,
1236,
504,
1600,
366,
272,
15991,
1600,
366,
272,
15991,
641,
33116,
628,
220,
220,
220,
14631,
64,
1600,
366,
397,
1600,
366,
39305,
1600,
366,
397,
10210,
1600,
366,
39305,
2934,
1600,
366,
39305,
4299,
1600,
366,
65,
1600,
366,
15630,
1600,
366,
65,
10210,
1600,
366,
15630,
2934,
1600,
366,
15630,
4299,
1600,
220,
198,
220,
220,
220,
220,
366,
15630,
4299,
70,
1600,
366,
6485,
1600,
366,
6485,
66,
1600,
366,
6485,
535,
1600,
366,
6485,
535,
1860,
33116,
628,
220,
220,
220,
14631,
5661,
1600,
366,
271,
1600,
366,
64,
1600,
366,
548,
1600,
366,
2865,
278,
1600,
366,
20688,
1600,
366,
4480,
1600,
366,
3919,
1600,
366,
11321,
1600,
366,
40290,
33116,
628,
220,
220,
220,
14631,
20888,
16,
2430,
20888,
17,
2430,
20888,
18,
2430,
20888,
19,
33116,
198,
220,
220,
220,
220,
628,
220,
220,
220,
1303,
770,
318,
422,
13606,
338,
787,
12,
260,
25636,
13,
417,
198,
220,
220,
220,
14631,
392,
1600,
366,
265,
1600,
366,
4598,
1600,
366,
437,
1600,
366,
1640,
1600,
366,
259,
1600,
366,
271,
1600,
366,
1662,
1600,
366,
1659,
1600,
366,
273,
1600,
366,
1904,
33116,
220,
220,
220,
220,
628,
220,
220,
220,
1303,
770,
318,
422,
13606,
338,
787,
12,
260,
25636,
13,
417,
198,
220,
220,
220,
14631,
17561,
1600,
366,
361,
1600,
366,
4514,
1600,
366,
1616,
9,
35379,
366,
1676,
70,
16,
1600,
366,
1676,
70,
17,
1600,
366,
1676,
4593,
1600,
198,
220,
220,
220,
220,
366,
40198,
1600,
366,
16939,
1600,
366,
21928,
12,
2118,
46214,
1600,
366,
21928,
12,
41194,
24197,
1600,
220,
198,
220,
220,
220,
220,
366,
21928,
12,
17497,
12,
41194,
24197,
1600,
366,
21928,
12,
15699,
12,
7890,
1600,
366,
403,
7972,
12,
35499,
1600,
220,
198,
220,
220,
220,
220,
366,
31448,
12,
7442,
1600,
366,
11659,
12,
35888,
33116,
198,
220,
220,
198,
220,
220,
220,
1303,
770,
318,
422,
13606,
338,
787,
12,
260,
25636,
13,
417,
198,
220,
220,
220,
14631,
397,
419,
1600,
366,
8937,
1600,
366,
13635,
1600,
366,
15526,
1600,
366,
18747,
1600,
198,
220,
220,
220,
220,
220,
366,
27471,
1600,
366,
2618,
1600,
366,
7442,
1600,
366,
9979,
415,
1600,
366,
32446,
533,
1600,
198,
220,
220,
220,
220,
220,
366,
40850,
1600,
366,
67,
12514,
1600,
366,
12894,
896,
1600,
366,
17772,
1600,
366,
1424,
361,
1600,
366,
13000,
1600,
198,
220,
220,
220,
220,
220,
366,
1069,
4516,
1600,
366,
37023,
1600,
366,
8818,
1600,
366,
41357,
1600,
366,
70,
2069,
1600,
198,
220,
220,
220,
220,
220,
366,
361,
1600,
366,
847,
82,
1600,
366,
10698,
1600,
366,
26268,
1600,
366,
4666,
1600,
366,
3605,
1600,
198,
220,
220,
220,
220,
220,
366,
8423,
1600,
366,
448,
1600,
366,
7266,
4906,
1600,
366,
26495,
1600,
366,
1050,
363,
2611,
1600,
198,
220,
220,
220,
220,
220,
366,
19734,
1600,
366,
1676,
771,
495,
1600,
366,
40225,
1600,
366,
9521,
1600,
366,
22105,
1600,
198,
220,
220,
220,
220,
220,
366,
2787,
1600,
366,
918,
1047,
1600,
366,
7783,
1600,
366,
50188,
1600,
366,
19738,
1600,
198,
220,
220,
220,
220,
220,
366,
25512,
378,
1600,
366,
35943,
1600,
366,
23705,
378,
1600,
366,
8524,
1600,
366,
4906,
1600,
198,
220,
220,
220,
220,
220,
366,
12518,
1600,
366,
4514,
1600,
366,
4480,
1600,
366,
87,
273,
8973,
198,
60,
198,
198,
1640,
256,
287,
5254,
220,
198,
220,
220,
220,
44872,
7203,
33407,
720,
83,
4943,
198,
220,
220,
220,
44872,
7,
15883,
62,
260,
25636,
7,
83,
4008,
198,
220,
220,
220,
44872,
3419,
198,
437,
198,
198,
2,
28,
198,
10879,
796,
938,
7,
41989,
8,
198,
40914,
796,
787,
62,
260,
25636,
7,
10879,
8,
198,
35235,
7,
10879,
8,
198,
35235,
7203,
260,
25636,
25,
3,
40914,
4943,
198,
9122,
62,
260,
25636,
7,
40914,
11,
10879,
8,
198,
46249
] | 2.198221 | 2,810 |
const olm_pomc_c = 1e5 # exploration parameter for MCTS
const olm_pomc_iterations = 300 # exploration parameter for MCTS
const olm_pomc_dt = 0.5 # exploration parameter for MCTS
mutable struct PomcNode
x::AbstractArray{Float64, 1}
u::AbstractArray{Float64, 1}
b::AbstractArray{Float64, 1}
adv_x::AbstractArray{Float64, 1}
c::pomdp.Collision
q::Float64
N::Int
Ns::Int
PomcNode() = new(Float64[], Float64[], Float64[], Float64[],
pomdp.Collision(), 0.0, 1, 1)
end
function plan_pomc(x::AbstractArray{Float64, 1}, b::AbstractArray{Float64, 1},
agent::Agent, adv_agent::Agent, world::World,
reward::Function, ctrl_d::Discretization, depth::Int)
#backup variables
adv_agent_controller! = adv_agent.controller!
adv_agent_custom = adv_agent.custom
x = copy(x)
x[1] = mod(x[1], world.road.path.S[end])
value = PomcNode()
value.x = x
value.b = b
value.adv_x = adv_agent.x
adv_agent.controller! = pomdp.adv_controller!
(c, _) = adv.predict_collision(adv_agent.x, agent.x, world)
value.c = c
node = GroupTree(value)
num_iterations = round(Int, olm_pomc_iterations / 4 * depth)
visited = Set{PomcNode}()
for i in 1:num_iterations
simulate_pomc(node, agent, adv_agent, world, reward,
ctrl_d, visited, depth)
end
us = Float64[]
qs = -Inf
ret = nothing
(adv_c, _) = predict_collision(adv_agent.x, x, world)
P = map(o -> reduce(+, map(i -> node.value.b[i] *
pomdp.P_adv(o, adv_c, pomdp.DRIVERS[i]),
1:length(pomdp.DRIVERS))), pomdp.ACTIONS)
for a in 1:length(node.next)
q = reduce(+, map(o -> node.next[a][o].value.q * P[o],
1:length(pomdp.ACTIONS)))
if q > qs
qs = q
us = node.next[a][1].value.u
ret = node
end
end
# revert variables
adv_agent.controller! = adv_agent_controller!
adv_agent.custom = adv_agent_custom
return (us, node)
end
function simulate_pomc(node::GroupTree, agent::Agent, adv_agent::Agent,
world::World, reward::Function, ctrl_d::Discretization,
visited::Set{PomcNode}, depth::Int)
if depth <= 0
return 0.0
end
node.value.x[1] = mod(node.value.x[1], world.road.path.S[end])
(adv_c, _) = predict_collision(node.value.adv_x, node.value.x, world)
if !(node.value in visited)
push!(visited, node.value)
# predict adv agent doing three possible actions
adv_NX = Array{Array{Float64, 1}, 1}(undef, length(pomdp.ACTIONS))
for o in pomdp.ACTIONS
oidx = Int(o)
adv_nx = copy(node.value.adv_x)
adv_agent.custom[3] = o
sim.advance!(sim.default_dynamics!, adv_nx, Pair(adv_agent, world), 0.0,
olm_pomc_dt, olm_h)
adv_nx[1] = mod(adv_nx[1], world.road.path.S[end])
adv_NX[oidx] = adv_nx
end
# allocate next level of the tree
la_len = ctrl_d.thr[end] * ctrl_d.pt[end] # number of actions to survey
node.next = Array{Array{GroupTree, 1}, 1}(undef, la_len)
for aidx in 1:la_len
node.next[aidx] = Array{GroupTree, 1}(undef, length(pomdp.ACTIONS))
end
for la in 0:(la_len - 1)
u = dis.ls2x(ctrl_d, la)
agent.custom = u
nx = copy(node.value.x)
sim.advance!(sim.default_dynamics!, nx, Pair(agent, world), 0.0,
olm_pomc_dt, olm_h)
nx[1] = mod(nx[1], world.road.path.S[end])
ra = 0.0
for o in pomdp.ACTIONS
oidx = Int(o)
adv_nx = adv_NX[oidx]
(nc, _) = adv.predict_collision(nx, adv_nx, world)
custom = agent.custom
agent.custom = nc
r = reward(node.value.x, u, nx, agent, world)
agent.custom = custom
bp = pomdp.update_belief(node.value.b, o, adv_c)
value = PomcNode()
value.x = nx
value.adv_x = adv_nx
value.u = u
value.b = bp
value.c = nc
value.q = r
next_node = GroupTree(value)
node.next[la + 1][oidx] = next_node
end
end
return 0.0
end
# probability of each observation
P = map(o -> reduce(+, map(i -> node.value.b[i] *
pomdp.P_adv(o, adv_c, pomdp.DRIVERS[i]),
1:length(pomdp.DRIVERS))), pomdp.ACTIONS)
# find the best action for value and exploration
as = -1
vs = -Inf
for a in 1:length(node.next)
# expected reward
ra = reduce(+, map(o -> node.next[a][o].value.q * P[o],
1:length(pomdp.ACTIONS)))
v = ra + olm_pomc_c * sqrt(log(node.value.Ns) /
reduce(+, map(o -> node.next[a][o].value.N,
1:length(pomdp.ACTIONS))))
if v > vs
vs = v
as = a
end
end
# sample a transition
cP = cumsum(P)
rnd = rand()
oidx = findfirst(i -> cP[i] >= rnd, 1:length(cP))
x = node.value.x
adv_nx = node.next[as][oidx].value.adv_x
u = node.next[as][oidx].value.u
nx = node.next[as][oidx].value.x
bp = node.next[as][oidx].value.b
(nc, _) = adv.predict_collision(nx, adv_nx, world)
custom = agent.custom
agent.custom = nc
r = reward(node.value.x, u, nx, agent, world)
agent.custom = custom
q = r + olm_gamma * simulate_pomc(node.next[as][oidx], agent, adv_agent,
world, reward, ctrl_d, visited, depth - 1)
# update Qsa for fuck's sake ---------------------------------------------- #
dq = (q - node.next[as][oidx].value.q)
node.next[as][oidx].value.q += dq / node.next[as][oidx].value.N
# this works best BY FAR
#node.next[as].value.q = max(q, node.next[as].value.q)
#alpha = 0.5
#Qsa = node.next[as].value.q
#Qsa = Qsa * (1 - alpha) + q * alpha
#node.next[as].value.q = Qsa
# ------------------------------------------------------------------------- #
node.next[as][oidx].value.N += 1
node.value.Ns += 1
return q
end
function rollout(x::AbstractArray{Float64, 1},
adv_x::AbstractArray{Float64, 1},
b::AbstractArray{Float64, 1},
agent::Agent, adv_agent::Agent,
world::World, reward::Function,
ctrl_d::Discretization, depth::Int)
if depth <= 0
return 0.0
end
return 0.0
# choose middle action, generally reasonable
end
function controller_pomc!(u::AbstractArray{Float64},
x::AbstractArray{Float64},
dx::AbstractArray{Float64},
agent_world::Pair{Agent, World}, t::Float64)
agent = agent_world.first
u[1] = agent.custom[1]
u[2] = agent.custom[2]
end
| [
9979,
25776,
76,
62,
79,
296,
66,
62,
66,
796,
352,
68,
20,
1303,
13936,
11507,
329,
337,
4177,
50,
198,
9979,
25776,
76,
62,
79,
296,
66,
62,
2676,
602,
796,
5867,
1303,
13936,
11507,
329,
337,
4177,
50,
198,
9979,
25776,
76,
62,
79,
296,
66,
62,
28664,
796,
657,
13,
20,
1303,
13936,
11507,
329,
337,
4177,
50,
198,
198,
76,
18187,
2878,
25505,
66,
19667,
198,
220,
2124,
3712,
23839,
19182,
90,
43879,
2414,
11,
352,
92,
198,
220,
334,
3712,
23839,
19182,
90,
43879,
2414,
11,
352,
92,
198,
220,
275,
3712,
23839,
19182,
90,
43879,
2414,
11,
352,
92,
198,
220,
1354,
62,
87,
3712,
23839,
19182,
90,
43879,
2414,
11,
352,
92,
198,
220,
269,
3712,
79,
296,
26059,
13,
22667,
1166,
198,
220,
10662,
3712,
43879,
2414,
198,
220,
399,
3712,
5317,
198,
220,
399,
82,
3712,
5317,
628,
220,
25505,
66,
19667,
3419,
796,
649,
7,
43879,
2414,
58,
4357,
48436,
2414,
58,
4357,
48436,
2414,
58,
4357,
48436,
2414,
58,
4357,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
279,
296,
26059,
13,
22667,
1166,
22784,
657,
13,
15,
11,
352,
11,
352,
8,
198,
437,
198,
198,
8818,
1410,
62,
79,
296,
66,
7,
87,
3712,
23839,
19182,
90,
43879,
2414,
11,
352,
5512,
275,
3712,
23839,
19182,
90,
43879,
2414,
11,
352,
5512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
5797,
3712,
36772,
11,
1354,
62,
25781,
3712,
36772,
11,
995,
3712,
10603,
11,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6721,
3712,
22203,
11,
269,
14859,
62,
67,
3712,
15642,
1186,
1634,
11,
6795,
3712,
5317,
8,
198,
220,
1303,
1891,
929,
9633,
198,
220,
1354,
62,
25781,
62,
36500,
0,
796,
1354,
62,
25781,
13,
36500,
0,
198,
220,
1354,
62,
25781,
62,
23144,
796,
1354,
62,
25781,
13,
23144,
628,
220,
2124,
796,
4866,
7,
87,
8,
198,
220,
2124,
58,
16,
60,
796,
953,
7,
87,
58,
16,
4357,
995,
13,
6344,
13,
6978,
13,
50,
58,
437,
12962,
628,
220,
1988,
796,
25505,
66,
19667,
3419,
198,
220,
1988,
13,
87,
796,
2124,
198,
220,
1988,
13,
65,
796,
275,
198,
220,
1988,
13,
32225,
62,
87,
796,
1354,
62,
25781,
13,
87,
198,
220,
1354,
62,
25781,
13,
36500,
0,
796,
279,
296,
26059,
13,
32225,
62,
36500,
0,
198,
220,
357,
66,
11,
4808,
8,
796,
1354,
13,
79,
17407,
62,
26000,
1166,
7,
32225,
62,
25781,
13,
87,
11,
5797,
13,
87,
11,
995,
8,
198,
220,
1988,
13,
66,
796,
269,
198,
220,
10139,
796,
4912,
27660,
7,
8367,
8,
628,
220,
997,
62,
2676,
602,
796,
2835,
7,
5317,
11,
25776,
76,
62,
79,
296,
66,
62,
2676,
602,
1220,
604,
1635,
6795,
8,
628,
220,
8672,
796,
5345,
90,
47,
296,
66,
19667,
92,
3419,
198,
220,
329,
1312,
287,
352,
25,
22510,
62,
2676,
602,
198,
220,
220,
220,
29308,
62,
79,
296,
66,
7,
17440,
11,
5797,
11,
1354,
62,
25781,
11,
995,
11,
6721,
11,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
14859,
62,
67,
11,
8672,
11,
6795,
8,
198,
220,
886,
628,
220,
514,
796,
48436,
2414,
21737,
198,
220,
10662,
82,
796,
532,
18943,
198,
220,
1005,
796,
2147,
198,
220,
357,
32225,
62,
66,
11,
4808,
8,
796,
4331,
62,
26000,
1166,
7,
32225,
62,
25781,
13,
87,
11,
2124,
11,
995,
8,
198,
220,
350,
796,
3975,
7,
78,
4613,
4646,
7,
28200,
3975,
7,
72,
4613,
10139,
13,
8367,
13,
65,
58,
72,
60,
1635,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
279,
296,
26059,
13,
47,
62,
32225,
7,
78,
11,
1354,
62,
66,
11,
279,
296,
26059,
13,
7707,
30194,
58,
72,
46570,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
352,
25,
13664,
7,
79,
296,
26059,
13,
7707,
30194,
4008,
828,
279,
296,
26059,
13,
10659,
11053,
8,
198,
220,
329,
257,
287,
352,
25,
13664,
7,
17440,
13,
19545,
8,
198,
220,
220,
220,
10662,
796,
4646,
7,
28200,
3975,
7,
78,
4613,
10139,
13,
19545,
58,
64,
7131,
78,
4083,
8367,
13,
80,
1635,
350,
58,
78,
4357,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
352,
25,
13664,
7,
79,
296,
26059,
13,
10659,
11053,
22305,
198,
220,
220,
220,
611,
10662,
1875,
10662,
82,
198,
220,
220,
220,
220,
220,
10662,
82,
796,
10662,
198,
220,
220,
220,
220,
220,
514,
796,
10139,
13,
19545,
58,
64,
7131,
16,
4083,
8367,
13,
84,
198,
220,
220,
220,
220,
220,
1005,
796,
10139,
198,
220,
220,
220,
886,
198,
220,
886,
628,
220,
1303,
34052,
9633,
198,
220,
1354,
62,
25781,
13,
36500,
0,
796,
1354,
62,
25781,
62,
36500,
0,
198,
220,
1354,
62,
25781,
13,
23144,
796,
1354,
62,
25781,
62,
23144,
628,
220,
1441,
357,
385,
11,
10139,
8,
198,
437,
198,
198,
8818,
29308,
62,
79,
296,
66,
7,
17440,
3712,
13247,
27660,
11,
5797,
3712,
36772,
11,
1354,
62,
25781,
3712,
36772,
11,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
995,
3712,
10603,
11,
6721,
3712,
22203,
11,
269,
14859,
62,
67,
3712,
15642,
1186,
1634,
11,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
8672,
3712,
7248,
90,
47,
296,
66,
19667,
5512,
6795,
3712,
5317,
8,
198,
220,
611,
6795,
19841,
657,
198,
220,
220,
220,
1441,
657,
13,
15,
198,
220,
886,
628,
220,
10139,
13,
8367,
13,
87,
58,
16,
60,
796,
953,
7,
17440,
13,
8367,
13,
87,
58,
16,
4357,
995,
13,
6344,
13,
6978,
13,
50,
58,
437,
12962,
198,
220,
357,
32225,
62,
66,
11,
4808,
8,
796,
4331,
62,
26000,
1166,
7,
17440,
13,
8367,
13,
32225,
62,
87,
11,
10139,
13,
8367,
13,
87,
11,
995,
8,
628,
220,
611,
5145,
7,
17440,
13,
8367,
287,
8672,
8,
198,
220,
220,
220,
4574,
0,
7,
4703,
863,
11,
10139,
13,
8367,
8,
628,
198,
220,
220,
220,
1303,
4331,
1354,
5797,
1804,
1115,
1744,
4028,
198,
220,
220,
220,
1354,
62,
45,
55,
796,
15690,
90,
19182,
90,
43879,
2414,
11,
352,
5512,
352,
92,
7,
917,
891,
11,
4129,
7,
79,
296,
26059,
13,
10659,
11053,
4008,
198,
220,
220,
220,
329,
267,
287,
279,
296,
26059,
13,
10659,
11053,
198,
220,
220,
220,
220,
220,
267,
312,
87,
796,
2558,
7,
78,
8,
628,
220,
220,
220,
220,
220,
1354,
62,
77,
87,
796,
4866,
7,
17440,
13,
8367,
13,
32225,
62,
87,
8,
198,
220,
220,
220,
220,
220,
1354,
62,
25781,
13,
23144,
58,
18,
60,
796,
267,
198,
220,
220,
220,
220,
220,
985,
13,
324,
19259,
0,
7,
14323,
13,
12286,
62,
67,
4989,
873,
28265,
1354,
62,
77,
87,
11,
39645,
7,
32225,
62,
25781,
11,
995,
828,
657,
13,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
25776,
76,
62,
79,
296,
66,
62,
28664,
11,
25776,
76,
62,
71,
8,
198,
220,
220,
220,
220,
220,
1354,
62,
77,
87,
58,
16,
60,
796,
953,
7,
32225,
62,
77,
87,
58,
16,
4357,
995,
13,
6344,
13,
6978,
13,
50,
58,
437,
12962,
628,
220,
220,
220,
220,
220,
1354,
62,
45,
55,
58,
1868,
87,
60,
796,
1354,
62,
77,
87,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
31935,
1306,
1241,
286,
262,
5509,
198,
220,
220,
220,
8591,
62,
11925,
796,
269,
14859,
62,
67,
13,
400,
81,
58,
437,
60,
1635,
269,
14859,
62,
67,
13,
457,
58,
437,
60,
1303,
1271,
286,
4028,
284,
5526,
198,
220,
220,
220,
10139,
13,
19545,
796,
15690,
90,
19182,
90,
13247,
27660,
11,
352,
5512,
352,
92,
7,
917,
891,
11,
8591,
62,
11925,
8,
198,
220,
220,
220,
329,
6133,
87,
287,
352,
25,
5031,
62,
11925,
198,
220,
220,
220,
220,
220,
10139,
13,
19545,
58,
1698,
87,
60,
796,
15690,
90,
13247,
27660,
11,
352,
92,
7,
917,
891,
11,
4129,
7,
79,
296,
26059,
13,
10659,
11053,
4008,
198,
220,
220,
220,
886,
198,
220,
220,
220,
220,
198,
220,
220,
220,
329,
8591,
287,
657,
37498,
5031,
62,
11925,
532,
352,
8,
198,
220,
220,
220,
220,
220,
334,
796,
595,
13,
7278,
17,
87,
7,
44755,
62,
67,
11,
8591,
8,
198,
220,
220,
220,
220,
220,
5797,
13,
23144,
796,
334,
198,
220,
220,
220,
220,
220,
299,
87,
796,
4866,
7,
17440,
13,
8367,
13,
87,
8,
198,
220,
220,
220,
220,
220,
985,
13,
324,
19259,
0,
7,
14323,
13,
12286,
62,
67,
4989,
873,
28265,
299,
87,
11,
39645,
7,
25781,
11,
995,
828,
657,
13,
15,
11,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
25776,
76,
62,
79,
296,
66,
62,
28664,
11,
25776,
76,
62,
71,
8,
198,
220,
220,
220,
220,
220,
299,
87,
58,
16,
60,
796,
953,
7,
77,
87,
58,
16,
4357,
995,
13,
6344,
13,
6978,
13,
50,
58,
437,
12962,
628,
220,
220,
220,
220,
220,
2179,
796,
657,
13,
15,
198,
220,
220,
220,
220,
220,
329,
267,
287,
279,
296,
26059,
13,
10659,
11053,
198,
220,
220,
220,
220,
220,
220,
220,
267,
312,
87,
796,
2558,
7,
78,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1354,
62,
77,
87,
796,
1354,
62,
45,
55,
58,
1868,
87,
60,
198,
220,
220,
220,
220,
220,
220,
220,
357,
10782,
11,
4808,
8,
796,
1354,
13,
79,
17407,
62,
26000,
1166,
7,
77,
87,
11,
1354,
62,
77,
87,
11,
995,
8,
628,
220,
220,
220,
220,
220,
220,
220,
2183,
796,
5797,
13,
23144,
198,
220,
220,
220,
220,
220,
220,
220,
5797,
13,
23144,
796,
299,
66,
198,
220,
220,
220,
220,
220,
220,
220,
374,
796,
6721,
7,
17440,
13,
8367,
13,
87,
11,
334,
11,
299,
87,
11,
5797,
11,
995,
8,
198,
220,
220,
220,
220,
220,
220,
220,
5797,
13,
23144,
796,
2183,
628,
220,
220,
220,
220,
220,
220,
220,
275,
79,
796,
279,
296,
26059,
13,
19119,
62,
6667,
2086,
7,
17440,
13,
8367,
13,
65,
11,
267,
11,
1354,
62,
66,
8,
628,
220,
220,
220,
220,
220,
220,
220,
1988,
796,
25505,
66,
19667,
3419,
198,
220,
220,
220,
220,
220,
220,
220,
1988,
13,
87,
796,
299,
87,
198,
220,
220,
220,
220,
220,
220,
220,
1988,
13,
32225,
62,
87,
796,
1354,
62,
77,
87,
198,
220,
220,
220,
220,
220,
220,
220,
1988,
13,
84,
796,
334,
198,
220,
220,
220,
220,
220,
220,
220,
1988,
13,
65,
796,
275,
79,
198,
220,
220,
220,
220,
220,
220,
220,
1988,
13,
66,
796,
299,
66,
198,
220,
220,
220,
220,
220,
220,
220,
1988,
13,
80,
796,
374,
628,
220,
220,
220,
220,
220,
220,
220,
1306,
62,
17440,
796,
4912,
27660,
7,
8367,
8,
198,
220,
220,
220,
220,
220,
220,
220,
10139,
13,
19545,
58,
5031,
1343,
352,
7131,
1868,
87,
60,
796,
1306,
62,
17440,
198,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
657,
13,
15,
198,
220,
886,
628,
220,
1303,
12867,
286,
1123,
13432,
198,
220,
350,
796,
3975,
7,
78,
4613,
4646,
7,
28200,
3975,
7,
72,
4613,
10139,
13,
8367,
13,
65,
58,
72,
60,
1635,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
279,
296,
26059,
13,
47,
62,
32225,
7,
78,
11,
1354,
62,
66,
11,
279,
296,
26059,
13,
7707,
30194,
58,
72,
46570,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
352,
25,
13664,
7,
79,
296,
26059,
13,
7707,
30194,
4008,
828,
279,
296,
26059,
13,
10659,
11053,
8,
198,
220,
1303,
1064,
262,
1266,
2223,
329,
1988,
290,
13936,
198,
220,
355,
796,
532,
16,
198,
220,
3691,
796,
532,
18943,
198,
220,
329,
257,
287,
352,
25,
13664,
7,
17440,
13,
19545,
8,
198,
220,
220,
220,
1303,
2938,
6721,
198,
220,
220,
220,
2179,
796,
4646,
7,
28200,
3975,
7,
78,
4613,
10139,
13,
19545,
58,
64,
7131,
78,
4083,
8367,
13,
80,
1635,
350,
58,
78,
4357,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
352,
25,
13664,
7,
79,
296,
26059,
13,
10659,
11053,
22305,
198,
220,
220,
220,
410,
796,
2179,
1343,
25776,
76,
62,
79,
296,
66,
62,
66,
1635,
19862,
17034,
7,
6404,
7,
17440,
13,
8367,
13,
47503,
8,
1220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4646,
7,
28200,
3975,
7,
78,
4613,
10139,
13,
19545,
58,
64,
7131,
78,
4083,
8367,
13,
45,
11,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
352,
25,
13664,
7,
79,
296,
26059,
13,
10659,
11053,
35514,
198,
220,
220,
220,
611,
410,
1875,
3691,
198,
220,
220,
220,
220,
220,
3691,
796,
410,
198,
220,
220,
220,
220,
220,
355,
796,
257,
198,
220,
220,
220,
886,
198,
220,
886,
628,
220,
1303,
6291,
257,
6801,
198,
220,
269,
47,
796,
269,
5700,
388,
7,
47,
8,
198,
220,
374,
358,
796,
43720,
3419,
198,
220,
267,
312,
87,
796,
1064,
11085,
7,
72,
4613,
269,
47,
58,
72,
60,
18189,
374,
358,
11,
352,
25,
13664,
7,
66,
47,
4008,
628,
220,
2124,
796,
10139,
13,
8367,
13,
87,
198,
220,
1354,
62,
77,
87,
796,
10139,
13,
19545,
58,
292,
7131,
1868,
87,
4083,
8367,
13,
32225,
62,
87,
198,
220,
334,
796,
10139,
13,
19545,
58,
292,
7131,
1868,
87,
4083,
8367,
13,
84,
198,
220,
299,
87,
796,
10139,
13,
19545,
58,
292,
7131,
1868,
87,
4083,
8367,
13,
87,
198,
220,
275,
79,
796,
10139,
13,
19545,
58,
292,
7131,
1868,
87,
4083,
8367,
13,
65,
628,
220,
357,
10782,
11,
4808,
8,
796,
1354,
13,
79,
17407,
62,
26000,
1166,
7,
77,
87,
11,
1354,
62,
77,
87,
11,
995,
8,
198,
220,
2183,
796,
5797,
13,
23144,
198,
220,
5797,
13,
23144,
796,
299,
66,
198,
220,
374,
796,
6721,
7,
17440,
13,
8367,
13,
87,
11,
334,
11,
299,
87,
11,
5797,
11,
995,
8,
198,
220,
5797,
13,
23144,
796,
2183,
628,
220,
10662,
796,
374,
1343,
25776,
76,
62,
28483,
2611,
1635,
29308,
62,
79,
296,
66,
7,
17440,
13,
19545,
58,
292,
7131,
1868,
87,
4357,
5797,
11,
1354,
62,
25781,
11,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
995,
11,
6721,
11,
269,
14859,
62,
67,
11,
8672,
11,
6795,
532,
352,
8,
628,
220,
1303,
4296,
1195,
11400,
329,
5089,
338,
11060,
20368,
26171,
1303,
198,
220,
288,
80,
796,
357,
80,
532,
10139,
13,
19545,
58,
292,
7131,
1868,
87,
4083,
8367,
13,
80,
8,
198,
220,
10139,
13,
19545,
58,
292,
7131,
1868,
87,
4083,
8367,
13,
80,
15853,
288,
80,
1220,
10139,
13,
19545,
58,
292,
7131,
1868,
87,
4083,
8367,
13,
45,
628,
220,
1303,
428,
2499,
1266,
11050,
39024,
198,
220,
1303,
17440,
13,
19545,
58,
292,
4083,
8367,
13,
80,
796,
3509,
7,
80,
11,
10139,
13,
19545,
58,
292,
4083,
8367,
13,
80,
8,
628,
220,
1303,
26591,
796,
657,
13,
20,
198,
220,
1303,
48,
11400,
796,
10139,
13,
19545,
58,
292,
4083,
8367,
13,
80,
198,
220,
1303,
48,
11400,
796,
1195,
11400,
1635,
357,
16,
532,
17130,
8,
1343,
10662,
1635,
17130,
198,
220,
1303,
17440,
13,
19545,
58,
292,
4083,
8367,
13,
80,
796,
1195,
11400,
198,
220,
1303,
16529,
45537,
1303,
628,
220,
10139,
13,
19545,
58,
292,
7131,
1868,
87,
4083,
8367,
13,
45,
15853,
352,
198,
220,
10139,
13,
8367,
13,
47503,
15853,
352,
628,
220,
1441,
10662,
198,
437,
198,
198,
8818,
38180,
7,
87,
3712,
23839,
19182,
90,
43879,
2414,
11,
352,
5512,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1354,
62,
87,
3712,
23839,
19182,
90,
43879,
2414,
11,
352,
5512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
275,
3712,
23839,
19182,
90,
43879,
2414,
11,
352,
5512,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
5797,
3712,
36772,
11,
1354,
62,
25781,
3712,
36772,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
995,
3712,
10603,
11,
6721,
3712,
22203,
11,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
269,
14859,
62,
67,
3712,
15642,
1186,
1634,
11,
6795,
3712,
5317,
8,
198,
220,
611,
6795,
19841,
657,
198,
220,
220,
220,
1441,
657,
13,
15,
198,
220,
886,
628,
220,
1441,
657,
13,
15,
628,
220,
1303,
3853,
3504,
2223,
11,
4143,
6397,
198,
437,
198,
198,
8818,
10444,
62,
79,
296,
66,
0,
7,
84,
3712,
23839,
19182,
90,
43879,
2414,
5512,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2124,
3712,
23839,
19182,
90,
43879,
2414,
5512,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
44332,
3712,
23839,
19182,
90,
43879,
2414,
5512,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
5797,
62,
6894,
3712,
47,
958,
90,
36772,
11,
2159,
5512,
256,
3712,
43879,
2414,
8,
198,
220,
5797,
796,
5797,
62,
6894,
13,
11085,
198,
220,
334,
58,
16,
60,
796,
5797,
13,
23144,
58,
16,
60,
198,
220,
334,
58,
17,
60,
796,
5797,
13,
23144,
58,
17,
60,
198,
437,
198
] | 2.060037 | 3,248 |
# Question 1
function findset(x::DisjointSetNode)
if (x !=x.p )
x.p = findset(x.p)
end
x.p
end
# Question 2
function link!(x,y)
if (x.rank > y.rank)
y.p=x
else (x.p =y)
if (x.rank == y.rank)
y.rank = y.rank + 1
end
end
end
function union!(x::DisjointSetNode,y::DisjointSetNode)
link!(findset(x),findset(y))
end
# Question 3
function hammingdistance(s1::String,s2::String)
teller = 0
for i = 1:length(s1)
if s1[i] != s2[i]
teller +=1
end
end
teller
end | [
2,
18233,
352,
198,
8818,
1064,
2617,
7,
87,
3712,
7279,
73,
1563,
7248,
19667,
8,
198,
220,
220,
220,
611,
357,
87,
14512,
87,
13,
79,
1267,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
13,
79,
796,
1064,
2617,
7,
87,
13,
79,
8,
198,
197,
437,
198,
197,
87,
13,
79,
198,
437,
198,
198,
2,
18233,
362,
198,
8818,
2792,
0,
7,
87,
11,
88,
8,
198,
220,
220,
220,
611,
357,
87,
13,
43027,
1875,
331,
13,
43027,
8,
198,
220,
220,
220,
220,
220,
220,
220,
331,
13,
79,
28,
87,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
357,
87,
13,
79,
796,
88,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
357,
87,
13,
43027,
6624,
331,
13,
43027,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
331,
13,
43027,
796,
331,
13,
43027,
1343,
352,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
6441,
0,
7,
87,
3712,
7279,
73,
1563,
7248,
19667,
11,
88,
3712,
7279,
73,
1563,
7248,
19667,
8,
198,
220,
220,
220,
2792,
0,
7,
19796,
2617,
7,
87,
828,
19796,
2617,
7,
88,
4008,
198,
437,
198,
198,
2,
18233,
513,
198,
8818,
8891,
2229,
30246,
7,
82,
16,
3712,
10100,
11,
82,
17,
3712,
10100,
8,
198,
220,
220,
220,
1560,
263,
796,
657,
198,
220,
220,
220,
329,
1312,
796,
352,
25,
13664,
7,
82,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
264,
16,
58,
72,
60,
14512,
264,
17,
58,
72,
60,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1560,
263,
15853,
16,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1560,
263,
198,
437
] | 1.823718 | 312 |
module GitHub
import Base.show
using Requests
import JSON
using HttpCommon
abstract GitHubType
abstract Owner <: GitHubType
# types
export User,
Organization,
Repo,
Issue,
HttpError,
AuthError,
StatsError
# methods
export authenticate,
set_api_endpoint,
set_web_endpoint,
user,
star,
unstar,
stargazers,
starred,
forks,
fork,
contributors,
commit_activity,
code_frequency,
participation,
punch_card,
collaborators,
iscollaborator,
add_collaborator,
remove_collaborator,
watchers,
watched,
watching,
watch,
unwatch,
followers,
following,
org,
orgs,
repo,
issue,
create_issue,
edit_issue,
issues
include("utils.jl")
include("endpoint.jl")
include("error.jl")
include("auth.jl")
include("users.jl")
include("organizations.jl")
include("repos.jl")
include("issues.jl")
include("starring.jl")
include("forks.jl")
include("statistics.jl")
include("collaborators.jl")
include("watching.jl")
end
| [
198,
21412,
21722,
198,
198,
11748,
7308,
13,
12860,
198,
198,
3500,
9394,
3558,
198,
11748,
19449,
198,
3500,
367,
29281,
17227,
628,
198,
397,
8709,
21722,
6030,
198,
397,
8709,
23853,
1279,
25,
21722,
6030,
628,
198,
2,
3858,
198,
39344,
11787,
11,
198,
220,
220,
220,
220,
220,
220,
12275,
11,
198,
220,
220,
220,
220,
220,
220,
1432,
78,
11,
198,
220,
220,
220,
220,
220,
220,
18232,
11,
198,
220,
220,
220,
220,
220,
220,
367,
29281,
12331,
11,
198,
220,
220,
220,
220,
220,
220,
26828,
12331,
11,
198,
220,
220,
220,
220,
220,
220,
20595,
12331,
198,
198,
2,
5050,
198,
39344,
8323,
5344,
11,
198,
220,
220,
220,
220,
220,
220,
900,
62,
15042,
62,
437,
4122,
11,
198,
220,
220,
220,
220,
220,
220,
900,
62,
12384,
62,
437,
4122,
11,
198,
220,
220,
220,
220,
220,
220,
2836,
11,
198,
220,
220,
220,
220,
220,
220,
3491,
11,
198,
220,
220,
220,
220,
220,
220,
555,
7364,
11,
198,
220,
220,
220,
220,
220,
220,
336,
853,
1031,
364,
11,
198,
220,
220,
220,
220,
220,
220,
31636,
11,
198,
220,
220,
220,
220,
220,
220,
43378,
11,
198,
220,
220,
220,
220,
220,
220,
15563,
11,
198,
220,
220,
220,
220,
220,
220,
20420,
11,
198,
220,
220,
220,
220,
220,
220,
4589,
62,
21797,
11,
198,
220,
220,
220,
220,
220,
220,
2438,
62,
35324,
11,
198,
220,
220,
220,
220,
220,
220,
10270,
11,
198,
220,
220,
220,
220,
220,
220,
10862,
62,
9517,
11,
198,
220,
220,
220,
220,
220,
220,
37886,
11,
198,
220,
220,
220,
220,
220,
220,
318,
26000,
4820,
1352,
11,
198,
220,
220,
220,
220,
220,
220,
751,
62,
26000,
4820,
1352,
11,
198,
220,
220,
220,
220,
220,
220,
4781,
62,
26000,
4820,
1352,
11,
198,
220,
220,
220,
220,
220,
220,
4383,
3533,
11,
198,
220,
220,
220,
220,
220,
220,
7342,
11,
198,
220,
220,
220,
220,
220,
220,
4964,
11,
198,
220,
220,
220,
220,
220,
220,
2342,
11,
198,
220,
220,
220,
220,
220,
220,
7379,
963,
11,
198,
220,
220,
220,
220,
220,
220,
10569,
11,
198,
220,
220,
220,
220,
220,
220,
1708,
11,
198,
220,
220,
220,
220,
220,
220,
8745,
11,
198,
220,
220,
220,
220,
220,
220,
8745,
82,
11,
198,
220,
220,
220,
220,
220,
220,
29924,
11,
198,
220,
220,
220,
220,
220,
220,
2071,
11,
198,
220,
220,
220,
220,
220,
220,
2251,
62,
21949,
11,
198,
220,
220,
220,
220,
220,
220,
4370,
62,
21949,
11,
198,
220,
220,
220,
220,
220,
220,
2428,
628,
198,
17256,
7203,
26791,
13,
20362,
4943,
198,
17256,
7203,
437,
4122,
13,
20362,
4943,
198,
17256,
7203,
18224,
13,
20362,
4943,
198,
17256,
7203,
18439,
13,
20362,
4943,
198,
17256,
7203,
18417,
13,
20362,
4943,
198,
17256,
7203,
9971,
4582,
13,
20362,
4943,
198,
17256,
7203,
260,
1930,
13,
20362,
4943,
198,
17256,
7203,
37165,
13,
20362,
4943,
198,
17256,
7203,
7364,
1806,
13,
20362,
4943,
198,
17256,
7203,
1640,
591,
13,
20362,
4943,
198,
17256,
7203,
14269,
3969,
13,
20362,
4943,
198,
17256,
7203,
26000,
4820,
2024,
13,
20362,
4943,
198,
17256,
7203,
50042,
13,
20362,
4943,
628,
198,
437,
628
] | 2.161111 | 540 |
export readgrid
if VERSION < v"0.4.0-dev+1419"
stringtoint(s) = int(s);
stringtofloat(s) = float(s);
else
stringtoint(s) = parse(Int64,s);
stringtofloat(s) = parse(Float64,s);
end
"""
**Summary:**
```
xyz,vert,pattr,ele,eattr,nd=function readgrid(fname::String)
```
**Description:**
Reads a *1D,2D,3D* topology grid from the file `fname` and stores data in
intermediate structure consisting of
- `xyz ::Array{Float64,2}` vertices positions
- `vert ::Array{Int64,2}` vertex numbers
- `pattr::Array{Int64,2}` vertex attributes
- `ele ::Array{Int64,2}` element connectivity
- `eattr::Array{Int64,2}` element attributes
- `nd::Int64` spatial dimension (<=topological dimension)
xyz,vert,pattr,ele,eattr,nd
as read from node and ele files. Can handle meshes generated with *triangle*,
*tetgen*, and the corresponding *1D* equivalent. The type of mesh (intervals,
triangles, tetraeders etc.) should be known by the caller of readgrid, when
creating the proper derived objects, i.e. topologies.
"""
function readgrid(fname::String)
# read vertex related stuff
fid = open(fname * ".node","r")
mesh_header = split(strip(readline(fid))) # read file header
nvert = stringtoint(mesh_header[1]) # number of vertices
nd = stringtoint(mesh_header[2]) # spatial dimension
npattr = stringtoint(mesh_header[3]) # number of point attributes
nidv = stringtoint(mesh_header[4]) # number of boundary markers
# allocate
xyz = zeros(Float64,nvert,nd)
vert = zeros(Int64, nvert, 2 )
pattr = zeros(Int64, nvert, nidv + npattr)
# read vertex positions, enumeration, and attributes
for i=1:nvert
data = split(strip(readline(fid)))
for j=1:nd
xyz[i,j] = stringtofloat(data[1+j])
end
vert[i,1] = i
vert[i,2] = stringtoint(data[1])
for j=1:nidv+npattr
pattr[i,j] = stringtoint(data[1+nd+j])
end
end
close(fid)
# read element related stuff
fid = open(fname * ".ele","r")
mesh_header = split(strip(readline(fid)))
nele = stringtoint(mesh_header[1]) # number of elements
ntop = stringtoint(mesh_header[2]) # number of entities per element
nide = stringtoint(mesh_header[3]) # number of element attributes
# allocate
ele = zeros(Int64,nele,ntop+1)
eattr = zeros(Int64,nele,nide)
# read element enumeration, connectivity and attributes
for k=1:nele
data = split(strip(readline(fid)))
for i=1:(ntop+1)
ele[k,i] = stringtoint(data[i])
end
for i=1:nide
eattr[k,i] = stringtoint(data[ntop+1+i])
end
end
close(fid)
return xyz,vert,pattr,ele,eattr,nd
end
# see http://userpages.umbc.edu/~squire/UCD_Form.htm
# readable with ParaView
function readgrid_avs(fname::String)
# read vertex related stuff
fid = open(fname * ".inp","r")
mesh_header = split(strip(readline(fid))) # read file header
nvert = stringtoint(mesh_header[1]) # number of vertices
nele = stringtoint(mesh_header[2]) # number of elements
npattr = stringtoint(mesh_header[3]) # number of point attributes
nide = stringtoint(mesh_header[4]) # number of element attributes
nmodel = stringtoint(mesh_header[5]) # model data (assume this is zero)
nd = 3 # spatial dimension
nide = 1 # overwrite above
ntop = 3
# allocate
xyz = zeros(Float64,nvert,nd)
vert = zeros(Int64, nvert, 2 )
# read vertex positions, enumeration, and attributes
for i=1:nvert
data = split(strip(readline(fid)))
for j=1:nd
xyz[i,j] = stringtofloat(data[1+j])
end
vert[i,1] = i
vert[i,2] = stringtoint(data[1])
end
ele = zeros(Int64,nele,4)
eattr = zeros(Int64,nele,nide) # ==1?
for k=1:nele
data = split(strip(readline(fid)))
if data[3]=="tri"
ele[k,1] = stringtoint(data[1])
for i=1:3
ele[k,1+i] = stringtoint(data[3+i])
end
for i=1:nide
eattr[k,i] = stringtoint(data[1+i])
end
end
end
# read from INP file?
pattr = zeros(Int64,nvert,1)
close(fid)
return xyz,vert,pattr,ele,eattr,nd
end
| [
39344,
1100,
25928,
198,
198,
361,
44156,
2849,
1279,
410,
1,
15,
13,
19,
13,
15,
12,
7959,
10,
1415,
1129,
1,
198,
220,
4731,
1462,
600,
7,
82,
8,
220,
220,
796,
493,
7,
82,
1776,
198,
220,
4731,
1462,
22468,
7,
82,
8,
796,
12178,
7,
82,
1776,
198,
17772,
198,
220,
4731,
1462,
600,
7,
82,
8,
220,
220,
796,
21136,
7,
5317,
2414,
11,
82,
1776,
198,
220,
4731,
1462,
22468,
7,
82,
8,
796,
21136,
7,
43879,
2414,
11,
82,
1776,
198,
437,
198,
198,
37811,
198,
1174,
22093,
25,
1174,
198,
15506,
63,
198,
2124,
45579,
11,
1851,
11,
79,
35226,
11,
11129,
11,
68,
35226,
11,
358,
28,
8818,
1100,
25928,
7,
69,
3672,
3712,
10100,
8,
198,
15506,
63,
198,
198,
1174,
11828,
25,
1174,
198,
198,
5569,
82,
257,
1635,
16,
35,
11,
17,
35,
11,
18,
35,
9,
1353,
1435,
10706,
422,
262,
2393,
4600,
69,
3672,
63,
290,
7000,
1366,
287,
198,
3849,
13857,
4645,
17747,
286,
198,
198,
12,
4600,
5431,
89,
220,
7904,
19182,
90,
43879,
2414,
11,
17,
92,
63,
9421,
1063,
6116,
198,
12,
4600,
1851,
7904,
19182,
90,
5317,
2414,
11,
17,
92,
63,
220,
220,
37423,
3146,
198,
12,
4600,
79,
35226,
3712,
19182,
90,
5317,
2414,
11,
17,
92,
63,
220,
220,
37423,
12608,
198,
12,
4600,
11129,
220,
7904,
19182,
90,
5317,
2414,
11,
17,
92,
63,
220,
220,
5002,
19843,
198,
12,
4600,
68,
35226,
3712,
19182,
90,
5317,
2414,
11,
17,
92,
63,
220,
220,
5002,
12608,
198,
12,
4600,
358,
3712,
5317,
2414,
63,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
21739,
15793,
38155,
28,
4852,
2770,
15793,
8,
628,
2124,
45579,
11,
1851,
11,
79,
35226,
11,
11129,
11,
68,
35226,
11,
358,
198,
198,
292,
1100,
422,
10139,
290,
9766,
3696,
13,
1680,
5412,
48754,
7560,
351,
1635,
28461,
9248,
25666,
198,
9,
83,
316,
5235,
25666,
290,
262,
11188,
1635,
16,
35,
9,
7548,
13,
383,
2099,
286,
19609,
357,
3849,
12786,
11,
198,
28461,
27787,
11,
28408,
430,
276,
364,
3503,
2014,
815,
307,
1900,
416,
262,
24955,
286,
1100,
25928,
11,
618,
198,
20123,
278,
262,
1774,
10944,
5563,
11,
1312,
13,
68,
13,
1353,
5823,
13,
198,
198,
37811,
198,
198,
8818,
1100,
25928,
7,
69,
3672,
3712,
10100,
8,
628,
220,
1303,
1100,
37423,
3519,
3404,
198,
220,
49909,
796,
1280,
7,
69,
3672,
1635,
27071,
17440,
2430,
81,
4943,
198,
220,
19609,
62,
25677,
796,
6626,
7,
36311,
7,
961,
1370,
7,
69,
312,
22305,
1303,
1100,
2393,
13639,
198,
220,
299,
1851,
220,
220,
220,
220,
220,
220,
796,
4731,
1462,
600,
7,
76,
5069,
62,
25677,
58,
16,
12962,
1303,
1271,
286,
9421,
1063,
198,
220,
299,
67,
220,
220,
220,
220,
220,
220,
220,
220,
220,
796,
4731,
1462,
600,
7,
76,
5069,
62,
25677,
58,
17,
12962,
1303,
21739,
15793,
198,
220,
45941,
35226,
220,
220,
220,
220,
220,
796,
4731,
1462,
600,
7,
76,
5069,
62,
25677,
58,
18,
12962,
1303,
1271,
286,
966,
12608,
198,
220,
299,
312,
85,
220,
220,
220,
220,
220,
220,
220,
796,
4731,
1462,
600,
7,
76,
5069,
62,
25677,
58,
19,
12962,
1303,
1271,
286,
18645,
19736,
628,
220,
1303,
31935,
198,
220,
2124,
45579,
220,
220,
796,
1976,
27498,
7,
43879,
2414,
11,
77,
1851,
11,
358,
8,
198,
220,
9421,
220,
796,
1976,
27498,
7,
5317,
2414,
11,
299,
1851,
11,
362,
1267,
198,
220,
279,
35226,
796,
1976,
27498,
7,
5317,
2414,
11,
299,
1851,
11,
299,
312,
85,
1343,
45941,
35226,
8,
628,
220,
1303,
1100,
37423,
6116,
11,
27056,
341,
11,
290,
12608,
198,
220,
329,
1312,
28,
16,
25,
77,
1851,
198,
220,
220,
220,
1366,
220,
220,
220,
220,
220,
220,
220,
220,
796,
6626,
7,
36311,
7,
961,
1370,
7,
69,
312,
22305,
198,
220,
220,
220,
329,
474,
28,
16,
25,
358,
198,
220,
220,
220,
220,
220,
2124,
45579,
58,
72,
11,
73,
60,
220,
220,
220,
220,
796,
4731,
1462,
22468,
7,
7890,
58,
16,
10,
73,
12962,
198,
220,
220,
220,
886,
198,
220,
220,
220,
9421,
58,
72,
11,
16,
60,
220,
220,
220,
796,
1312,
198,
220,
220,
220,
9421,
58,
72,
11,
17,
60,
220,
220,
220,
796,
4731,
1462,
600,
7,
7890,
58,
16,
12962,
198,
220,
220,
220,
329,
474,
28,
16,
25,
77,
312,
85,
10,
37659,
35226,
198,
220,
220,
220,
220,
220,
279,
35226,
58,
72,
11,
73,
60,
796,
4731,
1462,
600,
7,
7890,
58,
16,
10,
358,
10,
73,
12962,
198,
220,
220,
220,
886,
198,
220,
886,
198,
220,
1969,
7,
69,
312,
8,
628,
220,
1303,
1100,
5002,
3519,
3404,
198,
220,
49909,
796,
1280,
7,
69,
3672,
1635,
27071,
11129,
2430,
81,
4943,
198,
220,
19609,
62,
25677,
796,
6626,
7,
36311,
7,
961,
1370,
7,
69,
312,
22305,
198,
220,
497,
293,
220,
220,
796,
4731,
1462,
600,
7,
76,
5069,
62,
25677,
58,
16,
12962,
1303,
1271,
286,
4847,
198,
220,
299,
4852,
220,
220,
796,
4731,
1462,
600,
7,
76,
5069,
62,
25677,
58,
17,
12962,
1303,
1271,
286,
12066,
583,
5002,
198,
220,
299,
485,
220,
220,
796,
4731,
1462,
600,
7,
76,
5069,
62,
25677,
58,
18,
12962,
1303,
1271,
286,
5002,
12608,
628,
220,
1303,
31935,
198,
220,
9766,
220,
220,
220,
796,
1976,
27498,
7,
5317,
2414,
11,
710,
293,
11,
429,
404,
10,
16,
8,
198,
220,
304,
35226,
220,
796,
1976,
27498,
7,
5317,
2414,
11,
710,
293,
11,
77,
485,
8,
628,
220,
1303,
1100,
5002,
27056,
341,
11,
19843,
290,
12608,
198,
220,
329,
479,
28,
16,
25,
710,
293,
198,
220,
220,
220,
1366,
796,
6626,
7,
36311,
7,
961,
1370,
7,
69,
312,
22305,
198,
220,
220,
220,
329,
1312,
28,
16,
37498,
429,
404,
10,
16,
8,
198,
220,
220,
220,
220,
220,
9766,
58,
74,
11,
72,
60,
796,
4731,
1462,
600,
7,
7890,
58,
72,
12962,
198,
220,
220,
220,
886,
198,
220,
220,
220,
329,
1312,
28,
16,
25,
77,
485,
198,
220,
220,
220,
220,
220,
304,
35226,
58,
74,
11,
72,
60,
796,
4731,
1462,
600,
7,
7890,
58,
429,
404,
10,
16,
10,
72,
12962,
198,
220,
220,
220,
886,
198,
220,
886,
198,
220,
1969,
7,
69,
312,
8,
198,
220,
1441,
2124,
45579,
11,
1851,
11,
79,
35226,
11,
11129,
11,
68,
35226,
11,
358,
198,
198,
437,
198,
198,
2,
766,
2638,
1378,
7220,
31126,
13,
2178,
66,
13,
15532,
14,
93,
16485,
557,
14,
52,
8610,
62,
8479,
13,
19211,
198,
2,
31744,
351,
2547,
64,
7680,
198,
8818,
1100,
25928,
62,
615,
82,
7,
69,
3672,
3712,
10100,
8,
628,
220,
1303,
1100,
37423,
3519,
3404,
198,
220,
49909,
796,
1280,
7,
69,
3672,
1635,
27071,
259,
79,
2430,
81,
4943,
198,
220,
19609,
62,
25677,
796,
6626,
7,
36311,
7,
961,
1370,
7,
69,
312,
22305,
1303,
1100,
2393,
13639,
198,
220,
299,
1851,
220,
220,
220,
220,
220,
220,
796,
4731,
1462,
600,
7,
76,
5069,
62,
25677,
58,
16,
12962,
1303,
1271,
286,
9421,
1063,
198,
220,
497,
293,
220,
220,
220,
220,
220,
220,
220,
796,
4731,
1462,
600,
7,
76,
5069,
62,
25677,
58,
17,
12962,
1303,
1271,
286,
4847,
198,
220,
45941,
35226,
220,
220,
220,
220,
220,
796,
4731,
1462,
600,
7,
76,
5069,
62,
25677,
58,
18,
12962,
1303,
1271,
286,
966,
12608,
198,
220,
299,
485,
220,
220,
220,
220,
220,
220,
220,
796,
4731,
1462,
600,
7,
76,
5069,
62,
25677,
58,
19,
12962,
1303,
1271,
286,
5002,
12608,
198,
220,
299,
19849,
220,
220,
220,
220,
220,
796,
4731,
1462,
600,
7,
76,
5069,
62,
25677,
58,
20,
12962,
1303,
2746,
1366,
357,
562,
2454,
428,
318,
6632,
8,
628,
220,
299,
67,
220,
220,
796,
513,
1303,
21739,
15793,
198,
220,
299,
485,
796,
352,
1303,
49312,
2029,
198,
220,
299,
4852,
796,
513,
628,
220,
1303,
31935,
198,
220,
2124,
45579,
220,
220,
796,
1976,
27498,
7,
43879,
2414,
11,
77,
1851,
11,
358,
8,
198,
220,
9421,
220,
796,
1976,
27498,
7,
5317,
2414,
11,
299,
1851,
11,
362,
1267,
628,
220,
1303,
1100,
37423,
6116,
11,
27056,
341,
11,
290,
12608,
198,
220,
329,
1312,
28,
16,
25,
77,
1851,
198,
220,
220,
220,
1366,
220,
220,
220,
220,
220,
220,
220,
220,
796,
6626,
7,
36311,
7,
961,
1370,
7,
69,
312,
22305,
198,
220,
220,
220,
329,
474,
28,
16,
25,
358,
198,
220,
220,
220,
220,
220,
2124,
45579,
58,
72,
11,
73,
60,
220,
220,
220,
220,
796,
4731,
1462,
22468,
7,
7890,
58,
16,
10,
73,
12962,
198,
220,
220,
220,
886,
198,
220,
220,
220,
9421,
58,
72,
11,
16,
60,
220,
220,
220,
796,
1312,
198,
220,
220,
220,
9421,
58,
72,
11,
17,
60,
220,
220,
220,
796,
4731,
1462,
600,
7,
7890,
58,
16,
12962,
198,
220,
886,
628,
220,
9766,
220,
220,
220,
796,
1976,
27498,
7,
5317,
2414,
11,
710,
293,
11,
19,
8,
198,
220,
304,
35226,
220,
796,
1976,
27498,
7,
5317,
2414,
11,
710,
293,
11,
77,
485,
8,
1303,
6624,
16,
30,
628,
220,
329,
479,
28,
16,
25,
710,
293,
198,
220,
220,
220,
1366,
796,
6626,
7,
36311,
7,
961,
1370,
7,
69,
312,
22305,
198,
220,
220,
220,
611,
1366,
58,
18,
60,
855,
1,
28461,
1,
198,
220,
220,
220,
220,
220,
9766,
58,
74,
11,
16,
60,
796,
4731,
1462,
600,
7,
7890,
58,
16,
12962,
198,
220,
220,
220,
220,
220,
329,
1312,
28,
16,
25,
18,
198,
220,
220,
220,
220,
220,
220,
220,
9766,
58,
74,
11,
16,
10,
72,
60,
796,
4731,
1462,
600,
7,
7890,
58,
18,
10,
72,
12962,
198,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
329,
1312,
28,
16,
25,
77,
485,
198,
220,
220,
220,
220,
220,
220,
220,
304,
35226,
58,
74,
11,
72,
60,
796,
4731,
1462,
600,
7,
7890,
58,
16,
10,
72,
12962,
198,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
886,
628,
220,
1303,
1100,
422,
3268,
47,
2393,
30,
198,
220,
279,
35226,
796,
1976,
27498,
7,
5317,
2414,
11,
77,
1851,
11,
16,
8,
628,
220,
1969,
7,
69,
312,
8,
198,
220,
1441,
2124,
45579,
11,
1851,
11,
79,
35226,
11,
11129,
11,
68,
35226,
11,
358,
198,
198,
437,
198
] | 2.350827 | 1,753 |
"""
将三角切分的结果再进行切分
"""
module Refine
using ..Basics
export split_triangle, refine_triangles, find_adjs_by_adjoint
"""
将三角形重新切分成四个,可以用在直角三角形上
依旧保证出来的四个是直角三角形,且第一个角是直角
"""
@generated function split_triangle(rtri::Basics.AbstractTriangle{T}) where T
type2func = Dict(
:COM => Triangle,
:RT => RtTriangle,
:EQ => EqTriangle
)
func = type2func[T]
if T == :RT
return quote
#新的四个直角三角形的四个直角
rtvexs = Vector{Point2D}(undef, 4)
rtvexs[1] = rtri.vertex[1]
rtvexs[2] = middle_point(rtri.vertex[1], rtri.vertex[2])
rtvexs[3] = middle_point(rtri.vertex[2], rtri.vertex[3])
rtvexs[4] = middle_point(rtri.vertex[3], rtri.vertex[1])
#
newtris = Vector{Basics.AbstractTriangle{T}}(undef, 4)
newtris[1] = RtTriangle(rtvexs[2], rtvexs[3], rtvexs[1])
newtris[2] = RtTriangle(rtvexs[2], rtri.vertex[2], rtvexs[3])
newtris[3] = RtTriangle(rtvexs[4], rtvexs[3], rtri.vertex[3])
newtris[4] = RtTriangle(rtvexs[4], rtvexs[3], rtvexs[1])
return newtris
end
end
return quote
#新的四个直角三角形的四个直角
rtvexs = Vector{Point2D}(undef, 4)
rtvexs[1] = rtri.vertex[1]
rtvexs[2] = middle_point(rtri.vertex[1], rtri.vertex[2])
rtvexs[3] = middle_point(rtri.vertex[2], rtri.vertex[3])
rtvexs[4] = middle_point(rtri.vertex[3], rtri.vertex[1])
#
newtris = Vector{Basics.AbstractTriangle{T}}(undef, 4)
newtris[1] = ($func)(rtvexs[1], rtvexs[2], rtvexs[4])
newtris[2] = ($func)(rtvexs[2], rtri.vertex[2], rtvexs[3])
newtris[3] = ($func)(rtvexs[3], rtvexs[4], rtvexs[2])
newtris[4] = ($func)(rtvexs[4], rtvexs[3], rtri.vertex[3])
return newtris
end # end quote
end
"""
将一组的三角形细化
"""
function refine_triangles(ltris::Vector{T}) where T <: Basics.AbstractTriangle
newtris = Matrix{T}(undef, length(ltris), 4)
for (idx, tri) in enumerate(ltris)
newtris[idx, :] = split_triangle(tri)
end
newtris = reshape(newtris, length(ltris)*4)
return newtris
end
"""
判断一条边是不是某个三角形的
"""
macro isedge(edge, tri)
return esc(quote
topt1 = [vex - ($edge).pt1 for vex in ($tri).vertex]
topt1 = [sqrt(pt.x^2 + pt.y^2) for pt in topt1]
haspt1 = isapprox(minimum(topt1), 0., atol=1e-6)
topt2 = [vex - ($edge).pt2 for vex in ($tri).vertex]
topt2 = [sqrt(pt.x^2 + pt.y^2) for pt in topt2]
haspt2 = isapprox(minimum(topt2), 0., atol=1e-6)
haspt1 && haspt2
end)
end
"""
通过判断边是否重合来判断两个三角形是否挨在一起
"""
function find_adjs_by_adjoint(ltris::Vector{T}) where T <: Basics.AbstractTriangle
ladjs = Vector{Tuple{
Union{Missing, T}, Union{Missing, T}, Union{Missing, T}
}}(undef, length(ltris))
for idx in 1:1:length(ltris)
ladjs[idx] = (missing, missing, missing)
end
@Threads.threads for idx in 1:1:length(ltris)
tri = ltris[idx]
fadjs = Vector{Union{Missing, T}}(undef, 3)
fadjs[:] = [missing, missing, missing]
for (adjidx, adjtri) in enumerate(ltris)
if idx == adjidx
continue
end
if @isedge tri.edges[1] adjtri
fadjs[1] = adjtri
continue
end
if @isedge tri.edges[2] adjtri
fadjs[2] = adjtri
continue
end
if @isedge tri.edges[3] adjtri
fadjs[3] = adjtri
continue
end
end
ladjs[idx] = Tuple(fadjs)
end
return ladjs
end
end # end module
| [
37811,
198,
49546,
49011,
164,
100,
240,
26344,
229,
26344,
228,
21410,
163,
119,
241,
162,
252,
250,
37863,
235,
32573,
249,
26193,
234,
26344,
229,
26344,
228,
198,
37811,
198,
21412,
6524,
500,
198,
220,
220,
220,
220,
198,
3500,
11485,
15522,
873,
198,
198,
39344,
6626,
62,
28461,
9248,
11,
35139,
62,
28461,
27787,
11,
1064,
62,
324,
8457,
62,
1525,
62,
41255,
1563,
628,
198,
37811,
198,
49546,
49011,
164,
100,
240,
37605,
95,
34932,
235,
23877,
108,
26344,
229,
26344,
228,
22755,
238,
32368,
249,
10310,
103,
171,
120,
234,
20998,
107,
20015,
98,
18796,
101,
28839,
101,
33566,
112,
164,
100,
240,
49011,
164,
100,
240,
37605,
95,
41468,
198,
160,
122,
251,
33768,
100,
46479,
251,
46237,
223,
49035,
118,
30266,
98,
21410,
32368,
249,
10310,
103,
42468,
33566,
112,
164,
100,
240,
49011,
164,
100,
240,
37605,
95,
171,
120,
234,
10310,
242,
163,
105,
105,
31660,
10310,
103,
164,
100,
240,
42468,
33566,
112,
164,
100,
240,
198,
37811,
198,
31,
27568,
2163,
6626,
62,
28461,
9248,
7,
17034,
380,
3712,
15522,
873,
13,
23839,
14824,
9248,
90,
51,
30072,
810,
309,
198,
220,
220,
220,
2099,
17,
20786,
796,
360,
713,
7,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
9858,
5218,
33233,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
14181,
5218,
371,
83,
14824,
9248,
11,
198,
220,
220,
220,
220,
220,
220,
220,
1058,
36,
48,
5218,
412,
80,
14824,
9248,
198,
220,
220,
220,
1267,
198,
220,
220,
220,
25439,
796,
2099,
17,
20786,
58,
51,
60,
198,
220,
220,
220,
611,
309,
6624,
1058,
14181,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
9577,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
23877,
108,
21410,
32368,
249,
10310,
103,
33566,
112,
164,
100,
240,
49011,
164,
100,
240,
37605,
95,
21410,
32368,
249,
10310,
103,
33566,
112,
164,
100,
240,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
374,
83,
303,
34223,
796,
20650,
90,
12727,
17,
35,
92,
7,
917,
891,
11,
604,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
374,
83,
303,
34223,
58,
16,
60,
796,
374,
28461,
13,
332,
16886,
58,
16,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
374,
83,
303,
34223,
58,
17,
60,
796,
3504,
62,
4122,
7,
17034,
380,
13,
332,
16886,
58,
16,
4357,
374,
28461,
13,
332,
16886,
58,
17,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
374,
83,
303,
34223,
58,
18,
60,
796,
3504,
62,
4122,
7,
17034,
380,
13,
332,
16886,
58,
17,
4357,
374,
28461,
13,
332,
16886,
58,
18,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
374,
83,
303,
34223,
58,
19,
60,
796,
3504,
62,
4122,
7,
17034,
380,
13,
332,
16886,
58,
18,
4357,
374,
28461,
13,
332,
16886,
58,
16,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
2213,
271,
796,
20650,
90,
15522,
873,
13,
23839,
14824,
9248,
90,
51,
11709,
7,
917,
891,
11,
604,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
2213,
271,
58,
16,
60,
796,
371,
83,
14824,
9248,
7,
17034,
303,
34223,
58,
17,
4357,
374,
83,
303,
34223,
58,
18,
4357,
374,
83,
303,
34223,
58,
16,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
2213,
271,
58,
17,
60,
796,
371,
83,
14824,
9248,
7,
17034,
303,
34223,
58,
17,
4357,
374,
28461,
13,
332,
16886,
58,
17,
4357,
374,
83,
303,
34223,
58,
18,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
2213,
271,
58,
18,
60,
796,
371,
83,
14824,
9248,
7,
17034,
303,
34223,
58,
19,
4357,
374,
83,
303,
34223,
58,
18,
4357,
374,
28461,
13,
332,
16886,
58,
18,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
2213,
271,
58,
19,
60,
796,
371,
83,
14824,
9248,
7,
17034,
303,
34223,
58,
19,
4357,
374,
83,
303,
34223,
58,
18,
4357,
374,
83,
303,
34223,
58,
16,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1441,
649,
2213,
271,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
9577,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
23877,
108,
21410,
32368,
249,
10310,
103,
33566,
112,
164,
100,
240,
49011,
164,
100,
240,
37605,
95,
21410,
32368,
249,
10310,
103,
33566,
112,
164,
100,
240,
198,
220,
220,
220,
220,
220,
220,
220,
374,
83,
303,
34223,
796,
20650,
90,
12727,
17,
35,
92,
7,
917,
891,
11,
604,
8,
198,
220,
220,
220,
220,
220,
220,
220,
374,
83,
303,
34223,
58,
16,
60,
796,
374,
28461,
13,
332,
16886,
58,
16,
60,
198,
220,
220,
220,
220,
220,
220,
220,
374,
83,
303,
34223,
58,
17,
60,
796,
3504,
62,
4122,
7,
17034,
380,
13,
332,
16886,
58,
16,
4357,
374,
28461,
13,
332,
16886,
58,
17,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
374,
83,
303,
34223,
58,
18,
60,
796,
3504,
62,
4122,
7,
17034,
380,
13,
332,
16886,
58,
17,
4357,
374,
28461,
13,
332,
16886,
58,
18,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
374,
83,
303,
34223,
58,
19,
60,
796,
3504,
62,
4122,
7,
17034,
380,
13,
332,
16886,
58,
18,
4357,
374,
28461,
13,
332,
16886,
58,
16,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
198,
220,
220,
220,
220,
220,
220,
220,
649,
2213,
271,
796,
20650,
90,
15522,
873,
13,
23839,
14824,
9248,
90,
51,
11709,
7,
917,
891,
11,
604,
8,
198,
220,
220,
220,
220,
220,
220,
220,
649,
2213,
271,
58,
16,
60,
796,
7198,
20786,
5769,
17034,
303,
34223,
58,
16,
4357,
374,
83,
303,
34223,
58,
17,
4357,
374,
83,
303,
34223,
58,
19,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
649,
2213,
271,
58,
17,
60,
796,
7198,
20786,
5769,
17034,
303,
34223,
58,
17,
4357,
374,
28461,
13,
332,
16886,
58,
17,
4357,
374,
83,
303,
34223,
58,
18,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
649,
2213,
271,
58,
18,
60,
796,
7198,
20786,
5769,
17034,
303,
34223,
58,
18,
4357,
374,
83,
303,
34223,
58,
19,
4357,
374,
83,
303,
34223,
58,
17,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
649,
2213,
271,
58,
19,
60,
796,
7198,
20786,
5769,
17034,
303,
34223,
58,
19,
4357,
374,
83,
303,
34223,
58,
18,
4357,
374,
28461,
13,
332,
16886,
58,
18,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
649,
2213,
271,
198,
220,
220,
220,
886,
1303,
886,
9577,
198,
437,
628,
198,
37811,
198,
49546,
31660,
163,
119,
226,
21410,
49011,
164,
100,
240,
37605,
95,
163,
119,
228,
44293,
244,
198,
37811,
198,
8818,
35139,
62,
28461,
27787,
7,
75,
2213,
271,
3712,
38469,
90,
51,
30072,
810,
309,
1279,
25,
45884,
13,
23839,
14824,
9248,
198,
220,
220,
220,
649,
2213,
271,
796,
24936,
90,
51,
92,
7,
917,
891,
11,
4129,
7,
75,
2213,
271,
828,
604,
8,
198,
220,
220,
220,
329,
357,
312,
87,
11,
1333,
8,
287,
27056,
378,
7,
75,
2213,
271,
8,
198,
220,
220,
220,
220,
220,
220,
220,
649,
2213,
271,
58,
312,
87,
11,
1058,
60,
796,
6626,
62,
28461,
9248,
7,
28461,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
649,
2213,
271,
796,
27179,
1758,
7,
3605,
2213,
271,
11,
4129,
7,
75,
2213,
271,
27493,
19,
8,
198,
220,
220,
220,
1441,
649,
2213,
271,
198,
437,
628,
198,
37811,
198,
26344,
97,
23877,
255,
31660,
30266,
94,
164,
122,
117,
42468,
38834,
42468,
162,
253,
238,
10310,
103,
49011,
164,
100,
240,
37605,
95,
21410,
198,
37811,
198,
20285,
305,
318,
14907,
7,
14907,
11,
1333,
8,
198,
220,
220,
220,
1441,
3671,
7,
22708,
198,
220,
220,
220,
220,
220,
220,
220,
284,
457,
16,
796,
685,
303,
87,
532,
7198,
14907,
737,
457,
16,
329,
41548,
287,
7198,
28461,
737,
332,
16886,
60,
198,
220,
220,
220,
220,
220,
220,
220,
284,
457,
16,
796,
685,
31166,
17034,
7,
457,
13,
87,
61,
17,
1343,
42975,
13,
88,
61,
17,
8,
329,
42975,
287,
284,
457,
16,
60,
198,
220,
220,
220,
220,
220,
220,
220,
468,
457,
16,
796,
318,
1324,
13907,
7,
39504,
7,
4852,
83,
16,
828,
657,
1539,
379,
349,
28,
16,
68,
12,
21,
8,
198,
220,
220,
220,
220,
220,
220,
220,
284,
457,
17,
796,
685,
303,
87,
532,
7198,
14907,
737,
457,
17,
329,
41548,
287,
7198,
28461,
737,
332,
16886,
60,
198,
220,
220,
220,
220,
220,
220,
220,
284,
457,
17,
796,
685,
31166,
17034,
7,
457,
13,
87,
61,
17,
1343,
42975,
13,
88,
61,
17,
8,
329,
42975,
287,
284,
457,
17,
60,
198,
220,
220,
220,
220,
220,
220,
220,
468,
457,
17,
796,
318,
1324,
13907,
7,
39504,
7,
4852,
83,
17,
828,
657,
1539,
379,
349,
28,
16,
68,
12,
21,
8,
198,
220,
220,
220,
220,
220,
220,
220,
468,
457,
16,
11405,
468,
457,
17,
198,
220,
220,
220,
886,
8,
198,
437,
628,
198,
37811,
198,
34460,
248,
32573,
229,
26344,
97,
23877,
255,
164,
122,
117,
42468,
28938,
99,
34932,
235,
28938,
230,
30266,
98,
26344,
97,
23877,
255,
10310,
97,
10310,
103,
49011,
164,
100,
240,
37605,
95,
42468,
28938,
99,
162,
234,
101,
28839,
101,
31660,
164,
113,
115,
198,
37811,
198,
8818,
1064,
62,
324,
8457,
62,
1525,
62,
41255,
1563,
7,
75,
2213,
271,
3712,
38469,
90,
51,
30072,
810,
309,
1279,
25,
45884,
13,
23839,
14824,
9248,
198,
220,
220,
220,
9717,
8457,
796,
20650,
90,
51,
29291,
90,
198,
220,
220,
220,
220,
220,
220,
220,
4479,
90,
43730,
11,
309,
5512,
4479,
90,
43730,
11,
309,
5512,
4479,
90,
43730,
11,
309,
92,
198,
220,
220,
220,
34949,
7,
917,
891,
11,
4129,
7,
75,
2213,
271,
4008,
198,
220,
220,
220,
329,
4686,
87,
287,
352,
25,
16,
25,
13664,
7,
75,
2213,
271,
8,
198,
220,
220,
220,
220,
220,
220,
220,
9717,
8457,
58,
312,
87,
60,
796,
357,
45688,
11,
4814,
11,
4814,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
2488,
16818,
82,
13,
16663,
82,
329,
4686,
87,
287,
352,
25,
16,
25,
13664,
7,
75,
2213,
271,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1333,
796,
300,
2213,
271,
58,
312,
87,
60,
198,
220,
220,
220,
220,
220,
220,
220,
277,
324,
8457,
796,
20650,
90,
38176,
90,
43730,
11,
309,
11709,
7,
917,
891,
11,
513,
8,
198,
220,
220,
220,
220,
220,
220,
220,
277,
324,
8457,
58,
47715,
796,
685,
45688,
11,
4814,
11,
4814,
60,
198,
220,
220,
220,
220,
220,
220,
220,
329,
357,
41255,
312,
87,
11,
9224,
28461,
8,
287,
27056,
378,
7,
75,
2213,
271,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
4686,
87,
6624,
9224,
312,
87,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2555,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
2488,
1417,
469,
1333,
13,
276,
3212,
58,
16,
60,
9224,
28461,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
277,
324,
8457,
58,
16,
60,
796,
9224,
28461,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2555,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
2488,
1417,
469,
1333,
13,
276,
3212,
58,
17,
60,
9224,
28461,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
277,
324,
8457,
58,
17,
60,
796,
9224,
28461,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2555,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
2488,
1417,
469,
1333,
13,
276,
3212,
58,
18,
60,
9224,
28461,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
277,
324,
8457,
58,
18,
60,
796,
9224,
28461,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2555,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
9717,
8457,
58,
312,
87,
60,
796,
309,
29291,
7,
69,
324,
8457,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
9717,
8457,
198,
437,
628,
198,
198,
437,
1303,
886,
8265,
628
] | 1.659613 | 2,221 |
#!/usr/bin/env julia
# Copyright 2018 IBM Corp.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import SemanticFlowGraphs: CLI
function expand_paths(path::String)::Vector{String}
if isfile(path); [ path ]
elseif isdir(path); readdir(path)
else String[] end
end
cmds, cmd_args = CLI.parse(ARGS)
# XXX: Reduce load time by only importing extra packages as needed.
# Julia really needs a better solution to this problem...
if first(cmds) == "record"
paths = expand_paths(cmd_args["path"])
if any(endswith(path, ".py") for path in paths)
import PyCall
end
if any(endswith(path, ".R") for path in paths)
import RCall
end
end
CLI.invoke(cmds, cmd_args)
| [
2,
48443,
14629,
14,
8800,
14,
24330,
474,
43640,
198,
198,
2,
15069,
2864,
19764,
11421,
13,
198,
2,
198,
2,
49962,
739,
262,
24843,
13789,
11,
10628,
362,
13,
15,
357,
1169,
366,
34156,
15341,
198,
2,
345,
743,
407,
779,
428,
2393,
2845,
287,
11846,
351,
262,
13789,
13,
198,
2,
921,
743,
7330,
257,
4866,
286,
262,
13789,
379,
198,
2,
198,
2,
220,
220,
220,
220,
2638,
1378,
2503,
13,
43073,
13,
2398,
14,
677,
4541,
14,
43,
2149,
24290,
12,
17,
13,
15,
198,
2,
198,
2,
17486,
2672,
416,
9723,
1099,
393,
4987,
284,
287,
3597,
11,
3788,
198,
2,
9387,
739,
262,
13789,
318,
9387,
319,
281,
366,
1921,
3180,
1,
29809,
1797,
11,
198,
2,
42881,
34764,
11015,
6375,
7102,
49828,
11053,
3963,
15529,
509,
12115,
11,
2035,
4911,
393,
17142,
13,
198,
2,
4091,
262,
13789,
329,
262,
2176,
3303,
15030,
21627,
290,
198,
2,
11247,
739,
262,
13789,
13,
198,
198,
11748,
12449,
5109,
37535,
37065,
82,
25,
43749,
198,
198,
8818,
4292,
62,
6978,
82,
7,
6978,
3712,
10100,
2599,
25,
38469,
90,
10100,
92,
198,
220,
611,
318,
7753,
7,
6978,
1776,
685,
3108,
2361,
198,
220,
2073,
361,
318,
15908,
7,
6978,
1776,
1100,
15908,
7,
6978,
8,
198,
220,
2073,
10903,
21737,
886,
198,
437,
198,
198,
28758,
82,
11,
23991,
62,
22046,
796,
43749,
13,
29572,
7,
1503,
14313,
8,
198,
198,
2,
27713,
25,
44048,
3440,
640,
416,
691,
33332,
3131,
10392,
355,
2622,
13,
198,
2,
22300,
1107,
2476,
257,
1365,
4610,
284,
428,
1917,
986,
198,
361,
717,
7,
28758,
82,
8,
6624,
366,
22105,
1,
198,
220,
13532,
796,
4292,
62,
6978,
82,
7,
28758,
62,
22046,
14692,
6978,
8973,
8,
198,
220,
611,
597,
7,
437,
2032,
342,
7,
6978,
11,
27071,
9078,
4943,
329,
3108,
287,
13532,
8,
198,
220,
220,
220,
1330,
9485,
14134,
198,
220,
886,
198,
220,
611,
597,
7,
437,
2032,
342,
7,
6978,
11,
27071,
49,
4943,
329,
3108,
287,
13532,
8,
198,
220,
220,
220,
1330,
13987,
439,
198,
220,
886,
198,
437,
198,
198,
5097,
40,
13,
37669,
7,
28758,
82,
11,
23991,
62,
22046,
8,
198
] | 3.219178 | 365 |
module SummitBackgroundManager
using ..Ahorn, Maple
const placements = Ahorn.PlacementDict(
"Summit Background Manager" => Ahorn.EntityPlacement(
Maple.SummitBackgroundManager
)
)
function Ahorn.selection(entity::Maple.SummitBackgroundManager)
x, y = Ahorn.position(entity)
return Ahorn.Rectangle[Ahorn.Rectangle(x - 12, y - 12, 24, 24)]
end
Ahorn.render(ctx::Ahorn.Cairo.CairoContext, entity::Maple.SummitBackgroundManager, room::Maple.Room) = Ahorn.drawImage(ctx, Ahorn.Assets.summitBackgroundManager, -12, -12)
end | [
21412,
20014,
21756,
13511,
198,
198,
3500,
11485,
10910,
1211,
11,
21249,
198,
198,
9979,
21957,
3196,
796,
7900,
1211,
13,
3646,
5592,
35,
713,
7,
198,
220,
220,
220,
366,
13065,
2781,
25353,
9142,
1,
5218,
7900,
1211,
13,
32398,
3646,
5592,
7,
198,
220,
220,
220,
220,
220,
220,
220,
21249,
13,
13065,
2781,
21756,
13511,
198,
220,
220,
220,
1267,
198,
8,
198,
198,
8818,
7900,
1211,
13,
49283,
7,
26858,
3712,
13912,
293,
13,
13065,
2781,
21756,
13511,
8,
198,
220,
220,
220,
2124,
11,
331,
796,
7900,
1211,
13,
9150,
7,
26858,
8,
628,
220,
220,
220,
1441,
7900,
1211,
13,
45474,
9248,
58,
10910,
1211,
13,
45474,
9248,
7,
87,
532,
1105,
11,
331,
532,
1105,
11,
1987,
11,
1987,
15437,
198,
437,
198,
198,
10910,
1211,
13,
13287,
7,
49464,
3712,
10910,
1211,
13,
34,
18131,
13,
34,
18131,
21947,
11,
9312,
3712,
13912,
293,
13,
13065,
2781,
21756,
13511,
11,
2119,
3712,
13912,
293,
13,
41178,
8,
796,
7900,
1211,
13,
19334,
5159,
7,
49464,
11,
7900,
1211,
13,
8021,
1039,
13,
16345,
2781,
21756,
13511,
11,
532,
1065,
11,
532,
1065,
8,
198,
198,
437
] | 2.819588 | 194 |
##########################################################################
# Copyright 2017 Samuel Ridler.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
##########################################################################
# Dynamic Double Standard Model, with some modifications
# From paper: "A dynamic model and parallel tabu search heuristic for real-time ambulance relocation"
# Modifications (compared with original ddsm):
# - Added slack variables to coverage constraints, to avoid infeasibility. Cost of slack per unit is given by slackWeight.
# - Redeployment cost only depends on travel time, not on: ambulance history, avoiding round trips.
# initialise data relevant to move up
function initDdsm!(sim::Simulation;
coverFractionTargetT1::Float = 0.5, travelTimeCost::Float = 50.0, slackWeight::Float = 1e9,
coverTimeDemandPriorities::Vector{Priority} = [highPriority, lowPriority],
options::Dict{Symbol,Any} = Dict{Symbol,Any}())
# slackWeight is the weight to apply to slack variables, needs to be sufficiently large so that the slack variables are zero when possible
# coverTimeDemandPriorities[i] is demand priority for coverTimes[i], where coverTimes[1] and [2] are the targets for ddsm
@assert(0 <= coverFractionTargetT1 <= 1)
@assert(length(coverTimeDemandPriorities) == 2)
# initialise demand and demand coverage data if not already initialised
sim.demand.initialised || initDemand!(sim)
sim.demandCoverage.initialised || initDemandCoverage!(sim)
demand = sim.demand # shorthand
@assert(all([demandMode.rasterIndex for demandMode in demand.modes[demand.modeLookup[i,:]]] |> unique |> length == 1 for i = 1:demand.numSets)) # distribution of demand must be same for all demand priorities, in any given time period
# two target cover times for ddsm
coverTimes = [sim.demandCoverage.coverTimes[p] for p in coverTimeDemandPriorities]
@assert(length(coverTimes) == 2)
@assert(coverTimes[1] < coverTimes[2]) # change to warning?
# set options
ddsmd = sim.moveUpData.ddsmData # shorthand
ddsmd.options[:solver] = "cbc" # can be slower than glpk, but more reliable for some reason
ddsmd.options[:solver_args] = []
ddsmd.options[:solver_kwargs] = []
ddsmd.options[:v] = v"1"
ddsmd.options[:z_var] = true
ddsmd.options[:bin_tol] = 1e-5
merge!(ddsmd.options, Dict([:x_bin => true, :y11_bin => true, :y12_bin => true, :y2_bin => true]))
ddsmOptions!(sim, options)
ddsmd.coverFractionTargetT1 = coverFractionTargetT1
ddsmd.travelTimeCost = travelTimeCost
ddsmd.slackWeight = slackWeight
ddsmd.coverTimeDemandPriorities = coverTimeDemandPriorities # coverTimeDemandPriorities[i] is demand priority for coverTimes[i]
ddsmd.coverTimes = coverTimes
end
# change the options for ddsm
function ddsmOptions!(sim, options::Dict{Symbol,T}) where T <: Any
options = merge!(sim.moveUpData.ddsmData.options, options) # update options
@assert(in(options[:solver], ["cbc", "glpk", "gurobi"]))
@assert(typeof(options[:v]) == VersionNumber)
@assert(typeof(options[:z_var]) == Bool)
@assert(0 < options[:bin_tol] < 0.1)
if options[:solver] == "gurobi" try Gurobi; catch; options[:solver] = "cbc"; @warn("Failed to use Gurobi, using Cbc instead.") end end
# options[:v] == v"0" # do nothing, values should already be set in options if using this
options[:v] == v"1" && merge!(options, Dict([:x_bin => true, :y11_bin => true, :y12_bin => true, :y2_bin => true]))
options[:v] == v"2" && merge!(options, Dict([:x_bin => true, :y11_bin => true, :y12_bin => false, :y2_bin => false]))
options[:v] == v"3" && merge!(options, Dict([:x_bin => false, :y11_bin => true, :y12_bin => false, :y2_bin => false]))
options[:v] == v"4" && merge!(options, Dict([:x_bin => false, :y11_bin => true, :y12_bin => true, :y2_bin => true]))
if options[:y11_bin] == false && in(options[:solver], ["cbc", "glpk"]) @warn("Removing binary constraint for `y11` may not work with CBC or GLPK.") end
if options[:x_bin] == false && options[:solver] == "gurobi" @warn("Removing binary constraint for `x` may not work with Gurobi.") end
@assert(all(key -> haskey(options, key), [:x_bin, :y11_bin, :y12_bin, :y2_bin]))
if isdefined(sim, :backup) sim.backup.moveUpData.ddsmData.options = options end # to keep options if sim is reset
return options
end
function ddsmMoveUp(sim::Simulation)
@assert(sim.moveUpData.useMoveUp)
# shorthand:
@unpack ambulances, stations, numStations, demand = sim
currentTime = sim.time
ddsmd = sim.moveUpData.ddsmData
@unpack coverTimes, coverTimeDemandPriorities, coverFractionTargetT1, slackWeight, options = ddsmd
# get movable ambulances (movableAmbs)
ambMovable = [isAmbMovable(amb) for amb in ambulances]
movableAmbs = ambulances[ambMovable]
numMovableAmbs = length(movableAmbs)
if numMovableAmbs == 0
return moveUpNull()
end
# calculate travel time for each movable ambulance
ambToStationTimes = zeros(Float, numMovableAmbs, numStations) # ambToStationTimes[i,j] = time for movable ambulance i to travel to station j
for i = 1:numMovableAmbs
ambToStationTimes[i,:] = ambMoveUpTravelTimes!(sim, movableAmbs[i])
end
ambToStationCosts = ambToStationTimes * ddsmd.travelTimeCost
# get demand point coverage data for the two target cover times
# for coverTimes[ti], point j has demand pointDemands[ti][j] and is covered by stations pointStations[ti][j]
pointStations = [] # pointStations[ti][j] is coverTimes[ti]
pointDemands = []
numPoints = Int[] # can have different number of points (really point sets) for each cover time
demandPriorityArrivalRates = [getDemandMode!(demand, demandPriority, currentTime).arrivalRate for demandPriority in priorities]
for demandPriority in coverTimeDemandPriorities
# get demand point coverage data
pointsCoverageMode = getPointsCoverageMode!(sim, demandPriority, currentTime)
demandMode = getDemandMode!(demand, demandPriority, currentTime)
pointSetsDemands = getPointSetsDemands!(sim, demandPriority, currentTime; pointsCoverageMode = pointsCoverageMode) * demandMode.rasterMultiplier
pointSetsDemands *= sum(demandPriorityArrivalRates) / demandPriorityArrivalRates[Int(demandPriority)] # scale demand to be for all priorities, not just current demandPriority
push!(pointStations, pointsCoverageMode.stationSets)
push!(pointDemands, pointSetsDemands)
push!(numPoints, length(pointSetsDemands))
end
@assert(isapprox(sum(pointDemands[1]), sum(pointDemands[2])))
# could have it so that pointStations, pointDemands, and numPoints are only updated if the demandSet has changed since last query,
# but this may only shave off a couple of seconds per 100 sim days, which is not the current bottleneck
######################
# IP
# shorthand
a = numMovableAmbs
np = numPoints # np[ti] is number of points for coverTimes[i]
s = numStations
t = coverTimes
# using JuMP
model = Model()
solver = options[:solver] # shorthand
args = options[:solver_args] # shorthand
kwargs = options[:solver_kwargs] # shorthand
if solver == "cbc" set_optimizer(model, with_optimizer(Cbc.Optimizer, logLevel=0, args...; kwargs...))
elseif solver == "glpk" set_optimizer(model, with_optimizer(GLPK.Optimizer, args...; kwargs...))
elseif solver == "gurobi" @stdout_silent(set_optimizer(model, with_optimizer(Gurobi.Optimizer, OutputFlag=0, args...; kwargs...)))
end
m = model # shorthand
options[:x_bin] ? @variable(m, x[i=1:a,j=1:s], Bin) : @variable(m, 0 <= x[i=1:a,j=1:s] <= 1)
options[:y11_bin] ? @variable(m, y11[p=1:np[1]], Bin) : @variable(m, 0 <= y11[p=1:np[1]] <= 1)
options[:y12_bin] ? @variable(m, y12[p=1:np[1]], Bin) : @variable(m, 0 <= y12[p=1:np[1]] <= 1)
options[:y2_bin] ? @variable(m, y2[p=1:np[2]], Bin) : @variable(m, 0 <= y2[p=1:np[2]] <= 1)
# x[i,j] = 1 if ambulance: movableAmbs[i] should be moved to station: stations[j], 0 otherwise
# y11[p,k] = 1 if demand point p is covered at least once within t[1], 0 otherwise
# y12[p,k] = 1 if demand point p is covered at least twice within t[1], 0 otherwise
# y2[p] = 1 if demand point p is covered at least once within t[2], 0 otherwise
@variables(model, begin
(s1 >= 0) # slack
(s2 >= 0) # slack
end)
@constraints(model, begin
(ambAtOneStation[i=1:a], sum(x[i,:]) == 1) # each ambulance must be assigned to one station
(pointCoverOrderY1[p=1:np[1]], y11[p] >= y12[p]) # single coverage before double coverage; not needed for y2
(demandCoveredOnceT1, sum(y11[p] * pointDemands[1][p] for p=1:np[1]) + s1 >= coverFractionTargetT1 * sum(pointDemands[1])) # fraction of demand covered once within t[1]
(demandCoveredOnceT2, sum(y2[p] * pointDemands[2][p] for p=1:np[2]) + s2 >= sum(pointDemands[2])) # all demand covered once within t[2]
end)
if options[:z_var]
@variable(model, z[j=1:s], Int) # z[j] = number of ambulances to assign to station j
@constraints(model, begin
(stationAmbCount[j=1:s], z[j] == sum(x[:,j]))
(pointCoverCountY1[p=1:np[1]], y11[p] + y12[p] <= sum(z[pointStations[1][p]]))
(pointCoverCountY2[p=1:np[2]], y2[p] <= sum(z[pointStations[2][p]]))
end)
else
@constraints(model, begin
(pointCoverCountY1[p=1:np[1]], y11[p] + y12[p] <= sum(x[:,pointStations[1][p]]))
(pointCoverCountY2[p=1:np[2]], y2[p] <= sum(x[:,pointStations[2][p]]))
end)
end
@expressions(model, begin
demandCoveredTwiceT1, sum(y12[p] * pointDemands[1][p] for p=1:np[1])
totalAmbTravelCost, sum(x[i,j] * ambToStationCosts[i,j] for i=1:a, j=1:s)
slackCost, (s1 + s2) * slackWeight
end)
# solve
@objective(model, Max, demandCoveredTwiceT1 - totalAmbTravelCost - slackCost)
@stdout_silent optimize!(model)
@assert(termination_status(model) == MOI.OPTIMAL)
# get solution
vals = Dict()
vals[:x] = JuMP.value.(x)
vals[:y11] = JuMP.value.(y11)
vals[:y12] = JuMP.value.(y12)
vals[:y2] = JuMP.value.(y2)
sol = convert(Array{Bool,2}, round.(vals[:x]))
if checkMode
@assert(all(sum(sol, dims=2) .== 1)) # check constraint: ambAtOneStation
# check that values are binary
for sym in [:x, :y11, :y12, :y2]
err = maximum(abs.(vals[sym] - round.(vals[sym])))
@assert(err <= options[:bin_tol], (sym, err))
end
end
ambStations = [stations[findfirst(sol[i,:])] for i=1:a]
return movableAmbs, ambStations
end
| [
29113,
29113,
7804,
2235,
198,
2,
15069,
2177,
17100,
23223,
1754,
13,
198,
2,
198,
2,
49962,
739,
262,
24843,
13789,
11,
10628,
362,
13,
15,
357,
1169,
366,
34156,
15341,
198,
2,
345,
743,
407,
779,
428,
2393,
2845,
287,
11846,
351,
262,
13789,
13,
198,
2,
921,
743,
7330,
257,
4866,
286,
262,
13789,
379,
198,
2,
220,
220,
220,
220,
2638,
1378,
2503,
13,
43073,
13,
2398,
14,
677,
4541,
14,
43,
2149,
24290,
12,
17,
13,
15,
198,
2,
198,
2,
17486,
2672,
416,
9723,
1099,
393,
4987,
284,
287,
3597,
11,
3788,
198,
2,
9387,
739,
262,
13789,
318,
9387,
319,
281,
366,
1921,
3180,
1,
29809,
1797,
11,
198,
2,
42881,
34764,
11015,
6375,
7102,
49828,
11053,
3963,
15529,
509,
12115,
11,
2035,
4911,
393,
17142,
13,
198,
2,
4091,
262,
13789,
329,
262,
2176,
3303,
15030,
21627,
290,
198,
2,
11247,
739,
262,
13789,
13,
198,
29113,
29113,
7804,
2235,
198,
198,
2,
26977,
11198,
8997,
9104,
11,
351,
617,
19008,
198,
2,
3574,
3348,
25,
366,
32,
8925,
2746,
290,
10730,
7400,
84,
2989,
339,
27915,
329,
1103,
12,
2435,
22536,
35703,
1,
198,
198,
2,
3401,
6637,
357,
5589,
1144,
351,
2656,
49427,
5796,
2599,
198,
2,
532,
10687,
30740,
9633,
284,
5197,
17778,
11,
284,
3368,
1167,
30412,
2247,
13,
6446,
286,
30740,
583,
4326,
318,
1813,
416,
30740,
25844,
13,
198,
2,
532,
2297,
68,
1420,
434,
1575,
691,
8338,
319,
3067,
640,
11,
407,
319,
25,
22536,
2106,
11,
14928,
2835,
13229,
13,
198,
198,
2,
4238,
786,
1366,
5981,
284,
1445,
510,
198,
8818,
2315,
35,
67,
5796,
0,
7,
14323,
3712,
8890,
1741,
26,
198,
197,
9631,
37,
7861,
21745,
51,
16,
3712,
43879,
796,
657,
13,
20,
11,
3067,
7575,
13729,
3712,
43879,
796,
2026,
13,
15,
11,
30740,
25844,
3712,
43879,
796,
352,
68,
24,
11,
198,
197,
9631,
7575,
42782,
22442,
871,
3712,
38469,
90,
22442,
414,
92,
796,
685,
8929,
22442,
414,
11,
1877,
22442,
414,
4357,
198,
197,
25811,
3712,
35,
713,
90,
13940,
23650,
11,
7149,
92,
796,
360,
713,
90,
13940,
23650,
11,
7149,
92,
28955,
198,
197,
2,
30740,
25844,
318,
262,
3463,
284,
4174,
284,
30740,
9633,
11,
2476,
284,
307,
17338,
1588,
523,
326,
262,
30740,
9633,
389,
6632,
618,
1744,
198,
197,
2,
3002,
7575,
42782,
22442,
871,
58,
72,
60,
318,
3512,
8475,
329,
3002,
28595,
58,
72,
4357,
810,
3002,
28595,
58,
16,
60,
290,
685,
17,
60,
389,
262,
6670,
329,
49427,
5796,
198,
197,
198,
197,
31,
30493,
7,
15,
19841,
3002,
37,
7861,
21745,
51,
16,
19841,
352,
8,
198,
197,
31,
30493,
7,
13664,
7,
9631,
7575,
42782,
22442,
871,
8,
6624,
362,
8,
198,
197,
198,
197,
2,
4238,
786,
3512,
290,
3512,
5197,
1366,
611,
407,
1541,
4238,
1417,
198,
197,
14323,
13,
28550,
13,
36733,
1417,
8614,
2315,
42782,
0,
7,
14323,
8,
198,
197,
14323,
13,
28550,
7222,
1857,
13,
36733,
1417,
8614,
2315,
42782,
7222,
1857,
0,
7,
14323,
8,
198,
197,
28550,
796,
985,
13,
28550,
1303,
45883,
198,
197,
198,
197,
31,
30493,
7,
439,
26933,
28550,
19076,
13,
81,
1603,
15732,
329,
3512,
19076,
287,
3512,
13,
76,
4147,
58,
28550,
13,
14171,
8567,
929,
58,
72,
11,
25,
11907,
60,
930,
29,
3748,
930,
29,
4129,
6624,
352,
329,
1312,
796,
352,
25,
28550,
13,
22510,
50,
1039,
4008,
1303,
6082,
286,
3512,
1276,
307,
976,
329,
477,
3512,
15369,
11,
287,
597,
1813,
640,
2278,
198,
197,
198,
197,
2,
734,
2496,
3002,
1661,
329,
49427,
5796,
198,
197,
9631,
28595,
796,
685,
14323,
13,
28550,
7222,
1857,
13,
9631,
28595,
58,
79,
60,
329,
279,
287,
3002,
7575,
42782,
22442,
871,
60,
198,
197,
31,
30493,
7,
13664,
7,
9631,
28595,
8,
6624,
362,
8,
198,
197,
31,
30493,
7,
9631,
28595,
58,
16,
60,
1279,
3002,
28595,
58,
17,
12962,
1303,
1487,
284,
6509,
30,
198,
197,
198,
197,
2,
900,
3689,
198,
197,
1860,
5796,
67,
796,
985,
13,
21084,
4933,
6601,
13,
1860,
5796,
6601,
1303,
45883,
198,
197,
1860,
5796,
67,
13,
25811,
58,
25,
82,
14375,
60,
796,
366,
66,
15630,
1,
1303,
460,
307,
13611,
621,
1278,
79,
74,
11,
475,
517,
9314,
329,
617,
1738,
198,
197,
1860,
5796,
67,
13,
25811,
58,
25,
82,
14375,
62,
22046,
60,
796,
17635,
198,
197,
1860,
5796,
67,
13,
25811,
58,
25,
82,
14375,
62,
46265,
22046,
60,
796,
17635,
198,
197,
1860,
5796,
67,
13,
25811,
58,
25,
85,
60,
796,
410,
1,
16,
1,
198,
197,
1860,
5796,
67,
13,
25811,
58,
25,
89,
62,
7785,
60,
796,
2081,
198,
197,
1860,
5796,
67,
13,
25811,
58,
25,
8800,
62,
83,
349,
60,
796,
352,
68,
12,
20,
198,
197,
647,
469,
0,
7,
1860,
5796,
67,
13,
25811,
11,
360,
713,
26933,
25,
87,
62,
8800,
5218,
2081,
11,
1058,
88,
1157,
62,
8800,
5218,
2081,
11,
1058,
88,
1065,
62,
8800,
5218,
2081,
11,
1058,
88,
17,
62,
8800,
5218,
2081,
60,
4008,
198,
197,
1860,
5796,
29046,
0,
7,
14323,
11,
3689,
8,
198,
197,
198,
197,
1860,
5796,
67,
13,
9631,
37,
7861,
21745,
51,
16,
796,
3002,
37,
7861,
21745,
51,
16,
198,
197,
1860,
5796,
67,
13,
35927,
7575,
13729,
796,
3067,
7575,
13729,
198,
197,
1860,
5796,
67,
13,
6649,
441,
25844,
796,
30740,
25844,
198,
197,
1860,
5796,
67,
13,
9631,
7575,
42782,
22442,
871,
796,
3002,
7575,
42782,
22442,
871,
1303,
3002,
7575,
42782,
22442,
871,
58,
72,
60,
318,
3512,
8475,
329,
3002,
28595,
58,
72,
60,
198,
197,
1860,
5796,
67,
13,
9631,
28595,
796,
3002,
28595,
198,
437,
198,
198,
2,
1487,
262,
3689,
329,
49427,
5796,
198,
8818,
49427,
5796,
29046,
0,
7,
14323,
11,
3689,
3712,
35,
713,
90,
13940,
23650,
11,
51,
30072,
810,
309,
1279,
25,
4377,
198,
197,
25811,
796,
20121,
0,
7,
14323,
13,
21084,
4933,
6601,
13,
1860,
5796,
6601,
13,
25811,
11,
3689,
8,
1303,
4296,
3689,
198,
197,
198,
197,
31,
30493,
7,
259,
7,
25811,
58,
25,
82,
14375,
4357,
14631,
66,
15630,
1600,
366,
4743,
79,
74,
1600,
366,
70,
1434,
8482,
8973,
4008,
198,
197,
31,
30493,
7,
4906,
1659,
7,
25811,
58,
25,
85,
12962,
6624,
10628,
15057,
8,
198,
197,
31,
30493,
7,
4906,
1659,
7,
25811,
58,
25,
89,
62,
7785,
12962,
6624,
347,
970,
8,
198,
197,
31,
30493,
7,
15,
1279,
3689,
58,
25,
8800,
62,
83,
349,
60,
1279,
657,
13,
16,
8,
198,
197,
198,
197,
361,
3689,
58,
25,
82,
14375,
60,
6624,
366,
70,
1434,
8482,
1,
1949,
402,
1434,
8482,
26,
4929,
26,
3689,
58,
25,
82,
14375,
60,
796,
366,
66,
15630,
8172,
2488,
40539,
7203,
37,
6255,
284,
779,
402,
1434,
8482,
11,
1262,
327,
15630,
2427,
19570,
886,
886,
198,
197,
198,
197,
2,
3689,
58,
25,
85,
60,
6624,
410,
1,
15,
1,
1303,
466,
2147,
11,
3815,
815,
1541,
307,
900,
287,
3689,
611,
1262,
428,
198,
197,
25811,
58,
25,
85,
60,
6624,
410,
1,
16,
1,
11405,
20121,
0,
7,
25811,
11,
360,
713,
26933,
25,
87,
62,
8800,
5218,
2081,
11,
1058,
88,
1157,
62,
8800,
5218,
2081,
11,
1058,
88,
1065,
62,
8800,
5218,
2081,
11,
1058,
88,
17,
62,
8800,
5218,
2081,
60,
4008,
198,
197,
25811,
58,
25,
85,
60,
6624,
410,
1,
17,
1,
11405,
20121,
0,
7,
25811,
11,
360,
713,
26933,
25,
87,
62,
8800,
5218,
2081,
11,
1058,
88,
1157,
62,
8800,
5218,
2081,
11,
1058,
88,
1065,
62,
8800,
5218,
3991,
11,
1058,
88,
17,
62,
8800,
5218,
3991,
60,
4008,
198,
197,
25811,
58,
25,
85,
60,
6624,
410,
1,
18,
1,
11405,
20121,
0,
7,
25811,
11,
360,
713,
26933,
25,
87,
62,
8800,
5218,
3991,
11,
1058,
88,
1157,
62,
8800,
5218,
2081,
11,
1058,
88,
1065,
62,
8800,
5218,
3991,
11,
1058,
88,
17,
62,
8800,
5218,
3991,
60,
4008,
198,
197,
25811,
58,
25,
85,
60,
6624,
410,
1,
19,
1,
11405,
20121,
0,
7,
25811,
11,
360,
713,
26933,
25,
87,
62,
8800,
5218,
3991,
11,
1058,
88,
1157,
62,
8800,
5218,
2081,
11,
1058,
88,
1065,
62,
8800,
5218,
2081,
11,
1058,
88,
17,
62,
8800,
5218,
2081,
60,
4008,
198,
197,
361,
3689,
58,
25,
88,
1157,
62,
8800,
60,
6624,
3991,
11405,
287,
7,
25811,
58,
25,
82,
14375,
4357,
14631,
66,
15630,
1600,
366,
4743,
79,
74,
8973,
8,
2488,
40539,
7203,
8413,
5165,
13934,
32315,
329,
4600,
88,
1157,
63,
743,
407,
670,
351,
20244,
393,
10188,
40492,
19570,
886,
198,
197,
361,
3689,
58,
25,
87,
62,
8800,
60,
6624,
3991,
11405,
3689,
58,
25,
82,
14375,
60,
6624,
366,
70,
1434,
8482,
1,
2488,
40539,
7203,
8413,
5165,
13934,
32315,
329,
4600,
87,
63,
743,
407,
670,
351,
402,
1434,
8482,
19570,
886,
198,
197,
31,
30493,
7,
439,
7,
2539,
4613,
468,
2539,
7,
25811,
11,
1994,
828,
685,
25,
87,
62,
8800,
11,
1058,
88,
1157,
62,
8800,
11,
1058,
88,
1065,
62,
8800,
11,
1058,
88,
17,
62,
8800,
60,
4008,
198,
197,
198,
197,
361,
318,
23211,
7,
14323,
11,
1058,
1891,
929,
8,
985,
13,
1891,
929,
13,
21084,
4933,
6601,
13,
1860,
5796,
6601,
13,
25811,
796,
3689,
886,
1303,
284,
1394,
3689,
611,
985,
318,
13259,
198,
197,
198,
197,
7783,
3689,
198,
437,
198,
198,
8818,
49427,
5796,
21774,
4933,
7,
14323,
3712,
8890,
1741,
8,
198,
197,
31,
30493,
7,
14323,
13,
21084,
4933,
6601,
13,
1904,
21774,
4933,
8,
198,
197,
198,
197,
2,
45883,
25,
198,
197,
31,
403,
8002,
18907,
1817,
11,
8985,
11,
997,
1273,
602,
11,
3512,
796,
985,
198,
197,
14421,
7575,
796,
985,
13,
2435,
198,
197,
1860,
5796,
67,
796,
985,
13,
21084,
4933,
6601,
13,
1860,
5796,
6601,
198,
197,
31,
403,
8002,
3002,
28595,
11,
3002,
7575,
42782,
22442,
871,
11,
3002,
37,
7861,
21745,
51,
16,
11,
30740,
25844,
11,
3689,
796,
49427,
5796,
67,
198,
197,
198,
197,
2,
651,
1409,
540,
18907,
1817,
357,
76,
21985,
5840,
1443,
8,
198,
197,
4131,
44,
21985,
796,
685,
271,
35649,
44,
21985,
7,
4131,
8,
329,
4915,
287,
18907,
1817,
60,
198,
197,
76,
21985,
5840,
1443,
796,
18907,
1817,
58,
4131,
44,
21985,
60,
198,
197,
22510,
44,
21985,
5840,
1443,
796,
4129,
7,
76,
21985,
5840,
1443,
8,
198,
197,
198,
197,
361,
997,
44,
21985,
5840,
1443,
6624,
657,
198,
197,
197,
7783,
1445,
4933,
35067,
3419,
198,
197,
437,
198,
197,
198,
197,
2,
15284,
3067,
640,
329,
1123,
1409,
540,
22536,
198,
197,
4131,
2514,
12367,
28595,
796,
1976,
27498,
7,
43879,
11,
997,
44,
21985,
5840,
1443,
11,
997,
1273,
602,
8,
1303,
4915,
2514,
12367,
28595,
58,
72,
11,
73,
60,
796,
640,
329,
1409,
540,
22536,
1312,
284,
3067,
284,
4429,
474,
198,
197,
1640,
1312,
796,
352,
25,
22510,
44,
21985,
5840,
1443,
198,
197,
197,
4131,
2514,
12367,
28595,
58,
72,
11,
47715,
796,
4915,
21774,
4933,
33074,
28595,
0,
7,
14323,
11,
1409,
540,
5840,
1443,
58,
72,
12962,
198,
197,
437,
198,
197,
4131,
2514,
12367,
13729,
82,
796,
4915,
2514,
12367,
28595,
1635,
49427,
5796,
67,
13,
35927,
7575,
13729,
198,
197,
198,
197,
2,
651,
3512,
966,
5197,
1366,
329,
262,
734,
2496,
3002,
1661,
198,
197,
2,
329,
3002,
28595,
58,
20259,
4357,
966,
474,
468,
3512,
966,
11522,
1746,
58,
20259,
7131,
73,
60,
290,
318,
5017,
416,
8985,
966,
1273,
602,
58,
20259,
7131,
73,
60,
198,
197,
4122,
1273,
602,
796,
17635,
1303,
966,
1273,
602,
58,
20259,
7131,
73,
60,
318,
3002,
28595,
58,
20259,
60,
198,
197,
4122,
11522,
1746,
796,
17635,
198,
197,
22510,
40710,
796,
2558,
21737,
1303,
460,
423,
1180,
1271,
286,
2173,
357,
27485,
966,
5621,
8,
329,
1123,
3002,
640,
198,
197,
28550,
22442,
414,
3163,
43171,
49,
689,
796,
685,
1136,
42782,
19076,
0,
7,
28550,
11,
3512,
22442,
414,
11,
1459,
7575,
737,
283,
43171,
32184,
329,
3512,
22442,
414,
287,
15369,
60,
198,
197,
1640,
3512,
22442,
414,
287,
3002,
7575,
42782,
22442,
871,
198,
197,
197,
2,
651,
3512,
966,
5197,
1366,
198,
197,
197,
13033,
7222,
1857,
19076,
796,
651,
40710,
7222,
1857,
19076,
0,
7,
14323,
11,
3512,
22442,
414,
11,
1459,
7575,
8,
198,
197,
197,
28550,
19076,
796,
651,
42782,
19076,
0,
7,
28550,
11,
3512,
22442,
414,
11,
1459,
7575,
8,
198,
197,
197,
4122,
50,
1039,
11522,
1746,
796,
651,
12727,
50,
1039,
11522,
1746,
0,
7,
14323,
11,
3512,
22442,
414,
11,
1459,
7575,
26,
2173,
7222,
1857,
19076,
796,
2173,
7222,
1857,
19076,
8,
1635,
3512,
19076,
13,
81,
1603,
15205,
24705,
959,
198,
197,
197,
4122,
50,
1039,
11522,
1746,
1635,
28,
2160,
7,
28550,
22442,
414,
3163,
43171,
49,
689,
8,
1220,
3512,
22442,
414,
3163,
43171,
49,
689,
58,
5317,
7,
28550,
22442,
414,
15437,
1303,
5046,
3512,
284,
307,
329,
477,
15369,
11,
407,
655,
1459,
3512,
22442,
414,
198,
197,
197,
198,
197,
197,
14689,
0,
7,
4122,
1273,
602,
11,
2173,
7222,
1857,
19076,
13,
17529,
50,
1039,
8,
198,
197,
197,
14689,
0,
7,
4122,
11522,
1746,
11,
966,
50,
1039,
11522,
1746,
8,
198,
197,
197,
14689,
0,
7,
22510,
40710,
11,
4129,
7,
4122,
50,
1039,
11522,
1746,
4008,
198,
197,
437,
198,
197,
31,
30493,
7,
271,
1324,
13907,
7,
16345,
7,
4122,
11522,
1746,
58,
16,
46570,
2160,
7,
4122,
11522,
1746,
58,
17,
60,
22305,
198,
197,
2,
714,
423,
340,
523,
326,
966,
1273,
602,
11,
966,
11522,
1746,
11,
290,
997,
40710,
389,
691,
6153,
611,
262,
3512,
7248,
468,
3421,
1201,
938,
12405,
11,
198,
197,
2,
475,
428,
743,
691,
34494,
572,
257,
3155,
286,
4201,
583,
1802,
985,
1528,
11,
543,
318,
407,
262,
1459,
49936,
198,
197,
198,
197,
14468,
4242,
2235,
198,
197,
2,
6101,
198,
197,
198,
197,
2,
45883,
198,
197,
64,
796,
997,
44,
21985,
5840,
1443,
198,
197,
37659,
796,
997,
40710,
1303,
45941,
58,
20259,
60,
318,
1271,
286,
2173,
329,
3002,
28595,
58,
72,
60,
198,
197,
82,
796,
997,
1273,
602,
198,
197,
83,
796,
3002,
28595,
198,
197,
198,
197,
2,
1262,
12585,
7378,
198,
197,
19849,
796,
9104,
3419,
198,
197,
82,
14375,
796,
3689,
58,
25,
82,
14375,
60,
1303,
45883,
198,
197,
22046,
796,
3689,
58,
25,
82,
14375,
62,
22046,
60,
1303,
45883,
198,
197,
46265,
22046,
796,
3689,
58,
25,
82,
14375,
62,
46265,
22046,
60,
1303,
45883,
198,
197,
361,
1540,
332,
6624,
366,
66,
15630,
1,
900,
62,
40085,
7509,
7,
19849,
11,
351,
62,
40085,
7509,
7,
34,
15630,
13,
27871,
320,
7509,
11,
2604,
4971,
28,
15,
11,
26498,
986,
26,
479,
86,
22046,
986,
4008,
198,
197,
17772,
361,
1540,
332,
6624,
366,
4743,
79,
74,
1,
900,
62,
40085,
7509,
7,
19849,
11,
351,
62,
40085,
7509,
7,
8763,
40492,
13,
27871,
320,
7509,
11,
26498,
986,
26,
479,
86,
22046,
986,
4008,
198,
197,
17772,
361,
1540,
332,
6624,
366,
70,
1434,
8482,
1,
2488,
19282,
448,
62,
18217,
298,
7,
2617,
62,
40085,
7509,
7,
19849,
11,
351,
62,
40085,
7509,
7,
38,
1434,
8482,
13,
27871,
320,
7509,
11,
25235,
34227,
28,
15,
11,
26498,
986,
26,
479,
86,
22046,
986,
22305,
198,
197,
437,
198,
197,
198,
197,
76,
796,
2746,
1303,
45883,
198,
197,
25811,
58,
25,
87,
62,
8800,
60,
5633,
2488,
45286,
7,
76,
11,
2124,
58,
72,
28,
16,
25,
64,
11,
73,
28,
16,
25,
82,
4357,
20828,
8,
1058,
2488,
45286,
7,
76,
11,
657,
19841,
2124,
58,
72,
28,
16,
25,
64,
11,
73,
28,
16,
25,
82,
60,
19841,
352,
8,
198,
197,
25811,
58,
25,
88,
1157,
62,
8800,
60,
5633,
2488,
45286,
7,
76,
11,
331,
1157,
58,
79,
28,
16,
25,
37659,
58,
16,
60,
4357,
20828,
8,
1058,
2488,
45286,
7,
76,
11,
657,
19841,
331,
1157,
58,
79,
28,
16,
25,
37659,
58,
16,
11907,
19841,
352,
8,
198,
197,
25811,
58,
25,
88,
1065,
62,
8800,
60,
5633,
2488,
45286,
7,
76,
11,
331,
1065,
58,
79,
28,
16,
25,
37659,
58,
16,
60,
4357,
20828,
8,
1058,
2488,
45286,
7,
76,
11,
657,
19841,
331,
1065,
58,
79,
28,
16,
25,
37659,
58,
16,
11907,
19841,
352,
8,
198,
197,
25811,
58,
25,
88,
17,
62,
8800,
60,
5633,
2488,
45286,
7,
76,
11,
331,
17,
58,
79,
28,
16,
25,
37659,
58,
17,
60,
4357,
20828,
8,
1058,
2488,
45286,
7,
76,
11,
657,
19841,
331,
17,
58,
79,
28,
16,
25,
37659,
58,
17,
11907,
19841,
352,
8,
198,
197,
2,
2124,
58,
72,
11,
73,
60,
796,
352,
611,
22536,
25,
1409,
540,
5840,
1443,
58,
72,
60,
815,
307,
3888,
284,
4429,
25,
8985,
58,
73,
4357,
657,
4306,
198,
197,
2,
331,
1157,
58,
79,
11,
74,
60,
796,
352,
611,
3512,
966,
279,
318,
5017,
379,
1551,
1752,
1626,
256,
58,
16,
4357,
657,
4306,
198,
197,
2,
331,
1065,
58,
79,
11,
74,
60,
796,
352,
611,
3512,
966,
279,
318,
5017,
379,
1551,
5403,
1626,
256,
58,
16,
4357,
657,
4306,
198,
197,
2,
331,
17,
58,
79,
60,
796,
352,
611,
3512,
966,
279,
318,
5017,
379,
1551,
1752,
1626,
256,
58,
17,
4357,
657,
4306,
198,
197,
198,
197,
31,
25641,
2977,
7,
19849,
11,
2221,
198,
197,
197,
7,
82,
16,
18189,
657,
8,
1303,
30740,
198,
197,
197,
7,
82,
17,
18189,
657,
8,
1303,
30740,
198,
197,
437,
8,
198,
197,
198,
197,
31,
1102,
2536,
6003,
7,
19849,
11,
2221,
198,
197,
197,
7,
4131,
2953,
3198,
12367,
58,
72,
28,
16,
25,
64,
4357,
2160,
7,
87,
58,
72,
11,
25,
12962,
6624,
352,
8,
1303,
1123,
22536,
1276,
307,
8686,
284,
530,
4429,
198,
197,
197,
7,
4122,
27245,
18743,
56,
16,
58,
79,
28,
16,
25,
37659,
58,
16,
60,
4357,
331,
1157,
58,
79,
60,
18189,
331,
1065,
58,
79,
12962,
1303,
2060,
5197,
878,
4274,
5197,
26,
407,
2622,
329,
331,
17,
198,
197,
197,
7,
28550,
34,
2557,
7454,
51,
16,
11,
2160,
7,
88,
1157,
58,
79,
60,
1635,
966,
11522,
1746,
58,
16,
7131,
79,
60,
329,
279,
28,
16,
25,
37659,
58,
16,
12962,
1343,
264,
16,
18189,
3002,
37,
7861,
21745,
51,
16,
1635,
2160,
7,
4122,
11522,
1746,
58,
16,
60,
4008,
1303,
13390,
286,
3512,
5017,
1752,
1626,
256,
58,
16,
60,
198,
197,
197,
7,
28550,
34,
2557,
7454,
51,
17,
11,
2160,
7,
88,
17,
58,
79,
60,
1635,
966,
11522,
1746,
58,
17,
7131,
79,
60,
329,
279,
28,
16,
25,
37659,
58,
17,
12962,
1343,
264,
17,
18189,
2160,
7,
4122,
11522,
1746,
58,
17,
60,
4008,
1303,
477,
3512,
5017,
1752,
1626,
256,
58,
17,
60,
198,
197,
437,
8,
198,
197,
198,
197,
361,
3689,
58,
25,
89,
62,
7785,
60,
198,
197,
197,
31,
45286,
7,
19849,
11,
1976,
58,
73,
28,
16,
25,
82,
4357,
2558,
8,
1303,
1976,
58,
73,
60,
796,
1271,
286,
18907,
1817,
284,
8333,
284,
4429,
474,
198,
197,
197,
31,
1102,
2536,
6003,
7,
19849,
11,
2221,
198,
197,
197,
197,
7,
17529,
35649,
12332,
58,
73,
28,
16,
25,
82,
4357,
1976,
58,
73,
60,
6624,
2160,
7,
87,
58,
45299,
73,
60,
4008,
198,
197,
197,
197,
7,
4122,
27245,
12332,
56,
16,
58,
79,
28,
16,
25,
37659,
58,
16,
60,
4357,
331,
1157,
58,
79,
60,
1343,
331,
1065,
58,
79,
60,
19841,
2160,
7,
89,
58,
4122,
1273,
602,
58,
16,
7131,
79,
11907,
4008,
198,
197,
197,
197,
7,
4122,
27245,
12332,
56,
17,
58,
79,
28,
16,
25,
37659,
58,
17,
60,
4357,
331,
17,
58,
79,
60,
19841,
2160,
7,
89,
58,
4122,
1273,
602,
58,
17,
7131,
79,
11907,
4008,
198,
197,
197,
437,
8,
198,
197,
17772,
198,
197,
197,
31,
1102,
2536,
6003,
7,
19849,
11,
2221,
198,
197,
197,
197,
7,
4122,
27245,
12332,
56,
16,
58,
79,
28,
16,
25,
37659,
58,
16,
60,
4357,
331,
1157,
58,
79,
60,
1343,
331,
1065,
58,
79,
60,
19841,
2160,
7,
87,
58,
45299,
4122,
1273,
602,
58,
16,
7131,
79,
11907,
4008,
198,
197,
197,
197,
7,
4122,
27245,
12332,
56,
17,
58,
79,
28,
16,
25,
37659,
58,
17,
60,
4357,
331,
17,
58,
79,
60,
19841,
2160,
7,
87,
58,
45299,
4122,
1273,
602,
58,
17,
7131,
79,
11907,
4008,
198,
197,
197,
437,
8,
198,
197,
437,
198,
197,
198,
197,
31,
42712,
507,
7,
19849,
11,
2221,
198,
197,
197,
28550,
34,
2557,
5080,
501,
51,
16,
11,
2160,
7,
88,
1065,
58,
79,
60,
1635,
966,
11522,
1746,
58,
16,
7131,
79,
60,
329,
279,
28,
16,
25,
37659,
58,
16,
12962,
198,
197,
197,
23350,
35649,
33074,
13729,
11,
2160,
7,
87,
58,
72,
11,
73,
60,
1635,
4915,
2514,
12367,
13729,
82,
58,
72,
11,
73,
60,
329,
1312,
28,
16,
25,
64,
11,
474,
28,
16,
25,
82,
8,
198,
197,
197,
6649,
441,
13729,
11,
357,
82,
16,
1343,
264,
17,
8,
1635,
30740,
25844,
198,
197,
437,
8,
198,
197,
198,
197,
2,
8494,
198,
197,
31,
15252,
425,
7,
19849,
11,
5436,
11,
3512,
34,
2557,
5080,
501,
51,
16,
532,
2472,
35649,
33074,
13729,
532,
30740,
13729,
8,
198,
197,
31,
19282,
448,
62,
18217,
298,
27183,
0,
7,
19849,
8,
198,
197,
31,
30493,
7,
41382,
62,
13376,
7,
19849,
8,
6624,
13070,
40,
13,
3185,
51,
3955,
1847,
8,
198,
197,
198,
197,
2,
651,
4610,
198,
197,
12786,
796,
360,
713,
3419,
198,
197,
12786,
58,
25,
87,
60,
796,
12585,
7378,
13,
8367,
12195,
87,
8,
198,
197,
12786,
58,
25,
88,
1157,
60,
796,
12585,
7378,
13,
8367,
12195,
88,
1157,
8,
198,
197,
12786,
58,
25,
88,
1065,
60,
796,
12585,
7378,
13,
8367,
12195,
88,
1065,
8,
198,
197,
12786,
58,
25,
88,
17,
60,
796,
12585,
7378,
13,
8367,
12195,
88,
17,
8,
198,
197,
198,
197,
34453,
796,
10385,
7,
19182,
90,
33,
970,
11,
17,
5512,
2835,
12195,
12786,
58,
25,
87,
60,
4008,
198,
197,
198,
197,
361,
2198,
19076,
198,
197,
197,
31,
30493,
7,
439,
7,
16345,
7,
34453,
11,
5391,
82,
28,
17,
8,
764,
855,
352,
4008,
1303,
2198,
32315,
25,
4915,
2953,
3198,
12367,
198,
197,
197,
2,
2198,
326,
3815,
389,
13934,
198,
197,
197,
1640,
5659,
287,
685,
25,
87,
11,
1058,
88,
1157,
11,
1058,
88,
1065,
11,
1058,
88,
17,
60,
198,
197,
197,
197,
8056,
796,
5415,
7,
8937,
12195,
12786,
58,
37047,
60,
532,
2835,
12195,
12786,
58,
37047,
60,
22305,
198,
197,
197,
197,
31,
30493,
7,
8056,
19841,
3689,
58,
25,
8800,
62,
83,
349,
4357,
357,
37047,
11,
11454,
4008,
198,
197,
197,
437,
198,
197,
437,
198,
197,
198,
197,
4131,
1273,
602,
796,
685,
301,
602,
58,
19796,
11085,
7,
34453,
58,
72,
11,
25,
12962,
60,
329,
1312,
28,
16,
25,
64,
60,
198,
197,
198,
197,
7783,
1409,
540,
5840,
1443,
11,
4915,
1273,
602,
198,
437,
198
] | 2.753795 | 3,887 |
module Criterion
include("stats.jl")
include("analysis.jl")
include("types.jl")
include("measurement.jl")
include("environment.jl")
include("run.jl")
include("report.jl")
end
| [
21412,
10056,
28019,
198,
198,
17256,
7203,
34242,
13,
20362,
4943,
198,
17256,
7203,
20930,
13,
20362,
4943,
198,
17256,
7203,
19199,
13,
20362,
4943,
198,
17256,
7203,
1326,
5015,
434,
13,
20362,
4943,
198,
17256,
7203,
38986,
13,
20362,
4943,
198,
17256,
7203,
5143,
13,
20362,
4943,
198,
17256,
7203,
13116,
13,
20362,
4943,
198,
437,
198
] | 3.034483 | 58 |
#=
*** rewrite for import purposes ***
This is a resnet implementation by Deniz Yuret (https://github.com/denizyuret/Knet.jl/blob/master/examples/resnet/resnet.jl) with minor changes:
Copyright (c) 2015: Deniz Yuret.
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
julia resnet.jl image-file-or-url
This example implements the ResNet-50, ResNet-101 and ResNet-152 models from
'Deep Residual Learning for Image Regocnition', Kaiming He, Xiangyu Zhang,
Shaoqing Ren, Jian Sun, arXiv technical report 1512.03385, 2015.
* Paper url: https://arxiv.org/abs/1512.03385
* Project page: https://github.com/KaimingHe/deep-residual-networks
* MatConvNet weights used here: http://www.vlfeat.org/matconvnet/pretrained
=#
# mode, 0=>train, 1=>test
function resnet50(w,x,ms; mode=1)
# layer 1
conv1 = conv4(w[1],x; padding=3, stride=2) .+ w[2]
bn1 = batchnorm(w[3:4],conv1,ms; mode=mode)
pool1 = pool(bn1; window=3, stride=2)
# layer 2,3,4,5
r2 = reslayerx5(w[5:34], pool1, ms; strides=[1,1,1,1], mode=mode)
r3 = reslayerx5(w[35:73], r2, ms; mode=mode)
r4 = reslayerx5(w[74:130], r3, ms; mode=mode) # 5
r5 = reslayerx5(w[131:160], r4, ms; mode=mode)
# fully connected layer
pool5 = pool(r5; stride=1, window=7, mode=2)
fc1000 = w[161] * mat(pool5) .+ w[162]
end
# mode, 0=>train, 1=>test
function resnet101(w,x,ms; mode=1)
# layer 1
conv1 = reslayerx1(w[1:3],x,ms; padding=3, stride=2, mode=mode)
pool1 = pool(conv1; window=3, stride=2)
# layer 2,3,4,5
r2 = reslayerx5(w[4:33], pool1, ms; strides=[1,1,1,1], mode=mode)
r3 = reslayerx5(w[34:72], r2, ms; mode=mode)
r4 = reslayerx5(w[73:282], r3, ms; mode=mode)
r5 = reslayerx5(w[283:312], r4, ms; mode=mode)
# fully connected layer
pool5 = pool(r5; stride=1, window=7, mode=2)
fc1000 = w[313] * mat(pool5) .+ w[314]
end
# mode, 0=>train, 1=>test
function resnet152(w,x,ms; mode=1)
# layer 1
conv1 = reslayerx1(w[1:3],x,ms; padding=3, stride=2, mode=mode)
pool1 = pool(conv1; window=3, stride=2)
# layer 2,3,4,5
r2 = reslayerx5(w[4:33], pool1, ms; strides=[1,1,1,1], mode=mode)
r3 = reslayerx5(w[34:108], r2, ms; mode=mode)
r4 = reslayerx5(w[109:435], r3, ms; mode=mode)
r5 = reslayerx5(w[436:465], r4, ms; mode=mode)
# fully connected layer
pool5 = pool(r5; stride=1, window=7, mode=2)
fc1000 = w[466] * mat(pool5) .+ w[467]
end
# Batch Normalization Layer
# works both for convolutional and fully connected layers
# mode, 0=>train, 1=>test
function batchnorm(w, x, ms; mode=1, epsilon=1e-5)
mu, sigma = nothing, nothing
if mode == 0
d = ndims(x) == 4 ? (1,2,4) : (2,)
s = prod(size(x,d...))
mu = sum(x,d) / s
x0 = x .- mu
x1 = x0 .* x0
sigma = sqrt(epsilon + (sum(x1, d)) / s)
elseif mode == 1
mu = popfirst!(ms)
sigma = popfirst!(ms)
end
# we need value in backpropagation
push!(ms, value(mu), value(sigma))
xhat = (x.-mu) ./ sigma
return w[1] .* xhat .+ w[2]
end
function reslayerx0(w,x,ms; padding=0, stride=1, mode=1)
b = conv4(w[1],x; padding=padding, stride=stride)
bx = batchnorm(w[2:3],b,ms; mode=mode)
end
function reslayerx1(w,x,ms; padding=0, stride=1, mode=1)
relu.(reslayerx0(w,x,ms; padding=padding, stride=stride, mode=mode))
end
function reslayerx2(w,x,ms; pads=[0,1,0], strides=[1,1,1], mode=1)
ba = reslayerx1(w[1:3],x,ms; padding=pads[1], stride=strides[1], mode=mode)
bb = reslayerx1(w[4:6],ba,ms; padding=pads[2], stride=strides[2], mode=mode)
bc = reslayerx0(w[7:9],bb,ms; padding=pads[3], stride=strides[3], mode=mode)
end
function reslayerx3(w,x,ms; pads=[0,0,1,0], strides=[2,2,1,1], mode=1) # 12
a = reslayerx0(w[1:3],x,ms; stride=strides[1], padding=pads[1], mode=mode)
b = reslayerx2(w[4:12],x,ms; strides=strides[2:4], pads=pads[2:4], mode=mode)
relu.(a .+ b)
end
function reslayerx4(w,x,ms; pads=[0,1,0], strides=[1,1,1], mode=1)
relu.(x .+ reslayerx2(w,x,ms; pads=pads, strides=strides, mode=mode))
end
function reslayerx5(w,x,ms; strides=[2,2,1,1], mode=1)
x = reslayerx3(w[1:12],x,ms; strides=strides, mode=mode)
for k = 13:9:length(w)
x = reslayerx4(w[k:k+8],x,ms; mode=mode)
end
return x
end
function get_params(params, atype)
len = length(params["value"])
ws, ms = [], []
for k = 1:len
name = params["name"][k]
value = convert(Array{Float32}, params["value"][k])
if endswith(name, "moments")
push!(ms, reshape(value[:,1], (1,1,size(value,1),1)))
push!(ms, reshape(value[:,2], (1,1,size(value,1),1)))
elseif startswith(name, "bn")
push!(ws, reshape(value, (1,1,length(value),1)))
elseif startswith(name, "fc") && endswith(name, "filter")
push!(ws, transpose(reshape(value,(size(value,3),size(value,4)))))
elseif startswith(name, "conv") && endswith(name, "bias")
push!(ws, reshape(value, (1,1,length(value),1)))
else
push!(ws, value)
end
end
map(wi->convert(atype, wi), ws),
map(mi->convert(atype, mi), ms)
end
# This allows both non-interactive (shell command) and interactive calls like:
# $ julia vgg.jl cat.jpg
# julia> ResNet.main("cat.jpg")
#PROGRAM_FILE=="resnet.jl" && main(ARGS)
| [
2,
28,
198,
8162,
28183,
329,
1330,
4959,
17202,
198,
198,
1212,
318,
257,
581,
3262,
7822,
416,
5601,
528,
575,
495,
83,
357,
5450,
1378,
12567,
13,
785,
14,
6559,
528,
88,
495,
83,
14,
42,
3262,
13,
20362,
14,
2436,
672,
14,
9866,
14,
1069,
12629,
14,
411,
3262,
14,
411,
3262,
13,
20362,
8,
351,
4159,
2458,
25,
198,
198,
15269,
357,
66,
8,
1853,
25,
5601,
528,
575,
495,
83,
13,
198,
198,
5990,
3411,
318,
29376,
7520,
11,
1479,
286,
3877,
11,
284,
597,
1048,
16727,
257,
4866,
286,
428,
3788,
290,
3917,
10314,
3696,
357,
1169,
366,
25423,
12340,
284,
1730,
287,
262,
10442,
1231,
17504,
11,
1390,
1231,
17385,
262,
2489,
284,
779,
11,
4866,
11,
13096,
11,
20121,
11,
7715,
11,
14983,
11,
850,
43085,
11,
290,
14,
273,
3677,
9088,
286,
262,
10442,
11,
290,
284,
8749,
6506,
284,
4150,
262,
10442,
318,
30760,
284,
466,
523,
11,
2426,
284,
262,
1708,
3403,
25,
198,
198,
73,
43640,
581,
3262,
13,
20362,
2939,
12,
7753,
12,
273,
12,
6371,
198,
198,
1212,
1672,
23986,
262,
1874,
7934,
12,
1120,
11,
1874,
7934,
12,
8784,
290,
1874,
7934,
12,
17827,
4981,
422,
198,
6,
29744,
1874,
312,
723,
18252,
329,
7412,
3310,
420,
77,
653,
3256,
509,
1385,
278,
679,
11,
45641,
24767,
19439,
11,
198,
2484,
5488,
80,
278,
7152,
11,
40922,
3825,
11,
610,
55,
452,
6276,
989,
1315,
1065,
13,
44427,
5332,
11,
1853,
13,
198,
198,
9,
14962,
19016,
25,
3740,
1378,
283,
87,
452,
13,
2398,
14,
8937,
14,
1314,
1065,
13,
44427,
5332,
198,
9,
4935,
2443,
25,
3740,
1378,
12567,
13,
785,
14,
42,
1385,
278,
1544,
14,
22089,
12,
411,
312,
723,
12,
3262,
5225,
198,
9,
6550,
3103,
85,
7934,
19590,
973,
994,
25,
2638,
1378,
2503,
13,
85,
1652,
4098,
13,
2398,
14,
6759,
42946,
3262,
14,
5310,
13363,
198,
46249,
198,
198,
2,
4235,
11,
657,
14804,
27432,
11,
352,
14804,
9288,
198,
8818,
581,
3262,
1120,
7,
86,
11,
87,
11,
907,
26,
4235,
28,
16,
8,
198,
220,
220,
220,
1303,
7679,
352,
198,
220,
220,
220,
3063,
16,
220,
796,
3063,
19,
7,
86,
58,
16,
4357,
87,
26,
24511,
28,
18,
11,
33769,
28,
17,
8,
764,
10,
266,
58,
17,
60,
198,
220,
220,
220,
275,
77,
16,
220,
220,
220,
796,
15458,
27237,
7,
86,
58,
18,
25,
19,
4357,
42946,
16,
11,
907,
26,
4235,
28,
14171,
8,
198,
220,
220,
220,
5933,
16,
220,
796,
5933,
7,
9374,
16,
26,
4324,
28,
18,
11,
33769,
28,
17,
8,
628,
220,
220,
220,
1303,
7679,
362,
11,
18,
11,
19,
11,
20,
198,
220,
220,
220,
374,
17,
796,
581,
29289,
87,
20,
7,
86,
58,
20,
25,
2682,
4357,
5933,
16,
11,
13845,
26,
35002,
41888,
16,
11,
16,
11,
16,
11,
16,
4357,
4235,
28,
14171,
8,
198,
220,
220,
220,
374,
18,
796,
581,
29289,
87,
20,
7,
86,
58,
2327,
25,
4790,
4357,
374,
17,
11,
13845,
26,
4235,
28,
14171,
8,
198,
220,
220,
220,
374,
19,
796,
581,
29289,
87,
20,
7,
86,
58,
4524,
25,
12952,
4357,
374,
18,
11,
13845,
26,
4235,
28,
14171,
8,
1303,
642,
198,
220,
220,
220,
374,
20,
796,
581,
29289,
87,
20,
7,
86,
58,
22042,
25,
14198,
4357,
374,
19,
11,
13845,
26,
4235,
28,
14171,
8,
628,
220,
220,
220,
1303,
3938,
5884,
7679,
198,
220,
220,
220,
5933,
20,
220,
796,
5933,
7,
81,
20,
26,
33769,
28,
16,
11,
4324,
28,
22,
11,
4235,
28,
17,
8,
198,
220,
220,
220,
277,
66,
12825,
796,
266,
58,
25948,
60,
1635,
2603,
7,
7742,
20,
8,
764,
10,
266,
58,
25061,
60,
198,
437,
198,
198,
2,
4235,
11,
657,
14804,
27432,
11,
352,
14804,
9288,
198,
8818,
581,
3262,
8784,
7,
86,
11,
87,
11,
907,
26,
4235,
28,
16,
8,
198,
220,
220,
220,
1303,
7679,
352,
198,
220,
220,
220,
3063,
16,
796,
581,
29289,
87,
16,
7,
86,
58,
16,
25,
18,
4357,
87,
11,
907,
26,
24511,
28,
18,
11,
33769,
28,
17,
11,
4235,
28,
14171,
8,
198,
220,
220,
220,
5933,
16,
796,
5933,
7,
42946,
16,
26,
4324,
28,
18,
11,
33769,
28,
17,
8,
628,
220,
220,
220,
1303,
7679,
362,
11,
18,
11,
19,
11,
20,
198,
220,
220,
220,
374,
17,
796,
581,
29289,
87,
20,
7,
86,
58,
19,
25,
2091,
4357,
5933,
16,
11,
13845,
26,
35002,
41888,
16,
11,
16,
11,
16,
11,
16,
4357,
4235,
28,
14171,
8,
198,
220,
220,
220,
374,
18,
796,
581,
29289,
87,
20,
7,
86,
58,
2682,
25,
4761,
4357,
374,
17,
11,
13845,
26,
4235,
28,
14171,
8,
198,
220,
220,
220,
374,
19,
796,
581,
29289,
87,
20,
7,
86,
58,
4790,
25,
32568,
4357,
374,
18,
11,
13845,
26,
4235,
28,
14171,
8,
198,
220,
220,
220,
374,
20,
796,
581,
29289,
87,
20,
7,
86,
58,
30290,
25,
27970,
4357,
374,
19,
11,
13845,
26,
4235,
28,
14171,
8,
628,
220,
220,
220,
1303,
3938,
5884,
7679,
198,
220,
220,
220,
5933,
20,
220,
796,
5933,
7,
81,
20,
26,
33769,
28,
16,
11,
4324,
28,
22,
11,
4235,
28,
17,
8,
198,
220,
220,
220,
277,
66,
12825,
796,
266,
58,
25838,
60,
1635,
2603,
7,
7742,
20,
8,
764,
10,
266,
58,
33638,
60,
198,
437,
198,
198,
2,
4235,
11,
657,
14804,
27432,
11,
352,
14804,
9288,
198,
8818,
581,
3262,
17827,
7,
86,
11,
87,
11,
907,
26,
4235,
28,
16,
8,
198,
220,
220,
220,
1303,
7679,
352,
198,
220,
220,
220,
3063,
16,
796,
581,
29289,
87,
16,
7,
86,
58,
16,
25,
18,
4357,
87,
11,
907,
26,
24511,
28,
18,
11,
33769,
28,
17,
11,
4235,
28,
14171,
8,
198,
220,
220,
220,
5933,
16,
796,
5933,
7,
42946,
16,
26,
4324,
28,
18,
11,
33769,
28,
17,
8,
628,
220,
220,
220,
1303,
7679,
362,
11,
18,
11,
19,
11,
20,
198,
220,
220,
220,
374,
17,
796,
581,
29289,
87,
20,
7,
86,
58,
19,
25,
2091,
4357,
5933,
16,
11,
13845,
26,
35002,
41888,
16,
11,
16,
11,
16,
11,
16,
4357,
4235,
28,
14171,
8,
198,
220,
220,
220,
374,
18,
796,
581,
29289,
87,
20,
7,
86,
58,
2682,
25,
15711,
4357,
374,
17,
11,
13845,
26,
4235,
28,
14171,
8,
198,
220,
220,
220,
374,
19,
796,
581,
29289,
87,
20,
7,
86,
58,
14454,
25,
40064,
4357,
374,
18,
11,
13845,
26,
4235,
28,
14171,
8,
198,
220,
220,
220,
374,
20,
796,
581,
29289,
87,
20,
7,
86,
58,
43690,
25,
42018,
4357,
374,
19,
11,
13845,
26,
4235,
28,
14171,
8,
628,
220,
220,
220,
1303,
3938,
5884,
7679,
198,
220,
220,
220,
5933,
20,
220,
796,
5933,
7,
81,
20,
26,
33769,
28,
16,
11,
4324,
28,
22,
11,
4235,
28,
17,
8,
198,
220,
220,
220,
277,
66,
12825,
796,
266,
58,
42199,
60,
1635,
2603,
7,
7742,
20,
8,
764,
10,
266,
58,
24669,
60,
198,
437,
198,
198,
2,
347,
963,
14435,
1634,
34398,
198,
2,
2499,
1111,
329,
3063,
2122,
282,
290,
3938,
5884,
11685,
198,
2,
4235,
11,
657,
14804,
27432,
11,
352,
14804,
9288,
198,
8818,
15458,
27237,
7,
86,
11,
2124,
11,
13845,
26,
4235,
28,
16,
11,
304,
862,
33576,
28,
16,
68,
12,
20,
8,
198,
220,
220,
220,
38779,
11,
264,
13495,
796,
2147,
11,
2147,
198,
220,
220,
220,
611,
4235,
6624,
657,
198,
220,
220,
220,
220,
220,
220,
220,
288,
796,
299,
67,
12078,
7,
87,
8,
6624,
604,
5633,
357,
16,
11,
17,
11,
19,
8,
1058,
357,
17,
35751,
198,
220,
220,
220,
220,
220,
220,
220,
264,
796,
40426,
7,
7857,
7,
87,
11,
67,
986,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
38779,
796,
2160,
7,
87,
11,
67,
8,
1220,
264,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
15,
796,
2124,
764,
12,
38779,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
16,
796,
2124,
15,
764,
9,
2124,
15,
198,
220,
220,
220,
220,
220,
220,
220,
264,
13495,
796,
19862,
17034,
7,
538,
18217,
261,
1343,
357,
16345,
7,
87,
16,
11,
288,
4008,
1220,
264,
8,
198,
220,
220,
220,
2073,
361,
4235,
6624,
352,
198,
220,
220,
220,
220,
220,
220,
220,
38779,
796,
1461,
11085,
0,
7,
907,
8,
198,
220,
220,
220,
220,
220,
220,
220,
264,
13495,
796,
1461,
11085,
0,
7,
907,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1303,
356,
761,
1988,
287,
736,
22930,
363,
341,
198,
220,
220,
220,
4574,
0,
7,
907,
11,
1988,
7,
30300,
828,
1988,
7,
82,
13495,
4008,
198,
220,
220,
220,
2124,
5183,
796,
357,
87,
7874,
30300,
8,
24457,
264,
13495,
198,
220,
220,
220,
1441,
266,
58,
16,
60,
764,
9,
2124,
5183,
764,
10,
266,
58,
17,
60,
198,
437,
198,
198,
8818,
581,
29289,
87,
15,
7,
86,
11,
87,
11,
907,
26,
24511,
28,
15,
11,
33769,
28,
16,
11,
4235,
28,
16,
8,
198,
220,
220,
220,
275,
220,
796,
3063,
19,
7,
86,
58,
16,
4357,
87,
26,
24511,
28,
39231,
11,
33769,
28,
2536,
485,
8,
198,
220,
220,
220,
275,
87,
796,
15458,
27237,
7,
86,
58,
17,
25,
18,
4357,
65,
11,
907,
26,
4235,
28,
14171,
8,
198,
437,
198,
198,
8818,
581,
29289,
87,
16,
7,
86,
11,
87,
11,
907,
26,
24511,
28,
15,
11,
33769,
28,
16,
11,
4235,
28,
16,
8,
198,
220,
220,
220,
823,
84,
12195,
411,
29289,
87,
15,
7,
86,
11,
87,
11,
907,
26,
24511,
28,
39231,
11,
33769,
28,
2536,
485,
11,
4235,
28,
14171,
4008,
198,
437,
198,
198,
8818,
581,
29289,
87,
17,
7,
86,
11,
87,
11,
907,
26,
21226,
41888,
15,
11,
16,
11,
15,
4357,
35002,
41888,
16,
11,
16,
11,
16,
4357,
4235,
28,
16,
8,
198,
220,
220,
220,
26605,
796,
581,
29289,
87,
16,
7,
86,
58,
16,
25,
18,
4357,
87,
11,
907,
26,
24511,
28,
79,
5643,
58,
16,
4357,
33769,
28,
2536,
1460,
58,
16,
4357,
4235,
28,
14171,
8,
198,
220,
220,
220,
275,
65,
796,
581,
29289,
87,
16,
7,
86,
58,
19,
25,
21,
4357,
7012,
11,
907,
26,
24511,
28,
79,
5643,
58,
17,
4357,
33769,
28,
2536,
1460,
58,
17,
4357,
4235,
28,
14171,
8,
198,
220,
220,
220,
47125,
796,
581,
29289,
87,
15,
7,
86,
58,
22,
25,
24,
4357,
11848,
11,
907,
26,
24511,
28,
79,
5643,
58,
18,
4357,
33769,
28,
2536,
1460,
58,
18,
4357,
4235,
28,
14171,
8,
198,
437,
198,
198,
8818,
581,
29289,
87,
18,
7,
86,
11,
87,
11,
907,
26,
21226,
41888,
15,
11,
15,
11,
16,
11,
15,
4357,
35002,
41888,
17,
11,
17,
11,
16,
11,
16,
4357,
4235,
28,
16,
8,
1303,
1105,
198,
220,
220,
220,
257,
796,
581,
29289,
87,
15,
7,
86,
58,
16,
25,
18,
4357,
87,
11,
907,
26,
33769,
28,
2536,
1460,
58,
16,
4357,
24511,
28,
79,
5643,
58,
16,
4357,
4235,
28,
14171,
8,
198,
220,
220,
220,
275,
796,
581,
29289,
87,
17,
7,
86,
58,
19,
25,
1065,
4357,
87,
11,
907,
26,
35002,
28,
2536,
1460,
58,
17,
25,
19,
4357,
21226,
28,
79,
5643,
58,
17,
25,
19,
4357,
4235,
28,
14171,
8,
198,
220,
220,
220,
823,
84,
12195,
64,
764,
10,
275,
8,
198,
437,
198,
198,
8818,
581,
29289,
87,
19,
7,
86,
11,
87,
11,
907,
26,
21226,
41888,
15,
11,
16,
11,
15,
4357,
35002,
41888,
16,
11,
16,
11,
16,
4357,
4235,
28,
16,
8,
198,
220,
220,
220,
823,
84,
12195,
87,
764,
10,
581,
29289,
87,
17,
7,
86,
11,
87,
11,
907,
26,
21226,
28,
79,
5643,
11,
35002,
28,
2536,
1460,
11,
4235,
28,
14171,
4008,
198,
437,
198,
198,
8818,
581,
29289,
87,
20,
7,
86,
11,
87,
11,
907,
26,
35002,
41888,
17,
11,
17,
11,
16,
11,
16,
4357,
4235,
28,
16,
8,
198,
220,
220,
220,
2124,
796,
581,
29289,
87,
18,
7,
86,
58,
16,
25,
1065,
4357,
87,
11,
907,
26,
35002,
28,
2536,
1460,
11,
4235,
28,
14171,
8,
198,
220,
220,
220,
329,
479,
796,
1511,
25,
24,
25,
13664,
7,
86,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
796,
581,
29289,
87,
19,
7,
86,
58,
74,
25,
74,
10,
23,
4357,
87,
11,
907,
26,
4235,
28,
14171,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
2124,
198,
437,
198,
198,
8818,
651,
62,
37266,
7,
37266,
11,
379,
2981,
8,
198,
220,
220,
220,
18896,
796,
4129,
7,
37266,
14692,
8367,
8973,
8,
198,
220,
220,
220,
266,
82,
11,
13845,
796,
685,
4357,
17635,
198,
220,
220,
220,
329,
479,
796,
352,
25,
11925,
198,
220,
220,
220,
220,
220,
220,
220,
1438,
796,
42287,
14692,
3672,
1,
7131,
74,
60,
198,
220,
220,
220,
220,
220,
220,
220,
1988,
796,
10385,
7,
19182,
90,
43879,
2624,
5512,
42287,
14692,
8367,
1,
7131,
74,
12962,
628,
220,
220,
220,
220,
220,
220,
220,
611,
886,
2032,
342,
7,
3672,
11,
366,
32542,
658,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
907,
11,
27179,
1758,
7,
8367,
58,
45299,
16,
4357,
357,
16,
11,
16,
11,
7857,
7,
8367,
11,
16,
828,
16,
22305,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
907,
11,
27179,
1758,
7,
8367,
58,
45299,
17,
4357,
357,
16,
11,
16,
11,
7857,
7,
8367,
11,
16,
828,
16,
22305,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
361,
923,
2032,
342,
7,
3672,
11,
366,
9374,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
18504,
11,
27179,
1758,
7,
8367,
11,
357,
16,
11,
16,
11,
13664,
7,
8367,
828,
16,
22305,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
361,
923,
2032,
342,
7,
3672,
11,
366,
16072,
4943,
11405,
886,
2032,
342,
7,
3672,
11,
366,
24455,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
18504,
11,
1007,
3455,
7,
3447,
1758,
7,
8367,
11,
7,
7857,
7,
8367,
11,
18,
828,
7857,
7,
8367,
11,
19,
4008,
22305,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
361,
923,
2032,
342,
7,
3672,
11,
366,
42946,
4943,
11405,
886,
2032,
342,
7,
3672,
11,
366,
65,
4448,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
18504,
11,
27179,
1758,
7,
8367,
11,
357,
16,
11,
16,
11,
13664,
7,
8367,
828,
16,
22305,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
18504,
11,
1988,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
3975,
7,
37686,
3784,
1102,
1851,
7,
265,
2981,
11,
45967,
828,
266,
82,
828,
198,
220,
220,
220,
3975,
7,
11632,
3784,
1102,
1851,
7,
265,
2981,
11,
21504,
828,
13845,
8,
198,
437,
198,
198,
2,
770,
3578,
1111,
1729,
12,
3849,
5275,
357,
29149,
3141,
8,
290,
14333,
3848,
588,
25,
198,
2,
720,
474,
43640,
410,
1130,
13,
20362,
3797,
13,
9479,
198,
2,
474,
43640,
29,
1874,
7934,
13,
12417,
7203,
9246,
13,
9479,
4943,
198,
2,
4805,
7730,
24115,
62,
25664,
855,
1,
411,
3262,
13,
20362,
1,
11405,
1388,
7,
1503,
14313,
8,
198
] | 2.181438 | 2,629 |
#!/usr/bin/env julia
# Usage
#
# You can execute this file directly as long as the `julia` executable is
# in your PATH and if you are on a Linux/macOS system. If `julia` is not in
# your PATH or if you are on a Windows system, call Julia and explicitly
# provide this file as a command line parameter, e.g., `path/to/julia
# Trixi.jl/utils/install.jl`.
import Pkg
# Get Trixi root directory
trixi_root_dir = dirname(@__DIR__)
# Install Trixi dependencies
println("*"^80)
println("Installing dependencies for Trixi...")
Pkg.activate(trixi_root_dir)
Pkg.instantiate()
# Install Trixi2Img dependencies
println("*"^80)
println("Installing dependencies for Trixi2Img...")
Pkg.activate(joinpath(trixi_root_dir, "postprocessing", "pkg", "Trixi2Img"))
Pkg.instantiate()
# Install Trixi2Vtk dependencies
println("*"^80)
println("Installing dependencies for Trixi2Vtk...")
Pkg.activate(joinpath(trixi_root_dir, "postprocessing", "pkg", "Trixi2Vtk"))
Pkg.instantiate()
println("*"^80)
println("Done.")
| [
2,
48443,
14629,
14,
8800,
14,
24330,
474,
43640,
198,
198,
2,
29566,
198,
2,
198,
2,
921,
460,
12260,
428,
2393,
3264,
355,
890,
355,
262,
4600,
73,
43640,
63,
28883,
318,
198,
2,
287,
534,
46490,
290,
611,
345,
389,
319,
257,
7020,
14,
20285,
2640,
1080,
13,
1002,
4600,
73,
43640,
63,
318,
407,
287,
198,
2,
534,
46490,
393,
611,
345,
389,
319,
257,
3964,
1080,
11,
869,
22300,
290,
11777,
198,
2,
2148,
428,
2393,
355,
257,
3141,
1627,
11507,
11,
304,
13,
70,
1539,
4600,
6978,
14,
1462,
14,
73,
43640,
198,
2,
7563,
29992,
13,
20362,
14,
26791,
14,
17350,
13,
20362,
44646,
198,
198,
11748,
350,
10025,
198,
198,
2,
3497,
7563,
29992,
6808,
8619,
198,
83,
8609,
72,
62,
15763,
62,
15908,
796,
26672,
3672,
7,
31,
834,
34720,
834,
8,
198,
198,
2,
15545,
7563,
29992,
20086,
198,
35235,
7203,
9,
1,
61,
1795,
8,
198,
35235,
7203,
6310,
9221,
20086,
329,
7563,
29992,
9313,
8,
198,
47,
10025,
13,
39022,
7,
83,
8609,
72,
62,
15763,
62,
15908,
8,
198,
47,
10025,
13,
8625,
415,
9386,
3419,
198,
198,
2,
15545,
7563,
29992,
17,
3546,
70,
20086,
198,
35235,
7203,
9,
1,
61,
1795,
8,
198,
35235,
7203,
6310,
9221,
20086,
329,
7563,
29992,
17,
3546,
70,
9313,
8,
198,
47,
10025,
13,
39022,
7,
22179,
6978,
7,
83,
8609,
72,
62,
15763,
62,
15908,
11,
366,
7353,
36948,
1600,
366,
35339,
1600,
366,
51,
8609,
72,
17,
3546,
70,
48774,
198,
47,
10025,
13,
8625,
415,
9386,
3419,
198,
198,
2,
15545,
7563,
29992,
17,
53,
30488,
20086,
198,
35235,
7203,
9,
1,
61,
1795,
8,
198,
35235,
7203,
6310,
9221,
20086,
329,
7563,
29992,
17,
53,
30488,
9313,
8,
198,
47,
10025,
13,
39022,
7,
22179,
6978,
7,
83,
8609,
72,
62,
15763,
62,
15908,
11,
366,
7353,
36948,
1600,
366,
35339,
1600,
366,
51,
8609,
72,
17,
53,
30488,
48774,
198,
47,
10025,
13,
8625,
415,
9386,
3419,
198,
198,
35235,
7203,
9,
1,
61,
1795,
8,
198,
35235,
7203,
45677,
19570,
198
] | 2.878963 | 347 |
# Energy
function NbodyEnergy(u,Gm)
"""
Nbody problem Hamiltonian (Cartesian Coordinates)
"""
dim=2
nbody=length(Gm)
@inbounds begin
x = view(u,1:7) # x
y = view(u,8:14) # y
v = view(u,15:21) # x′
w = view(u,22:28) # y′
H=zero(eltype(u))
P=zero(eltype(u))
for i in 1:nbody
H+=Gm[i]*(v[i]*v[i]+w[i]*w[i])
for j in i+1:nbody
r = ((x[i]-x[j])^2+(y[i]-y[j])^2)^(1/2)
P+=(Gm[i]/r)*Gm[j]
end
end
return(H/2-P)
end
end
# OdeProblem
function f(du,u,p,t)
@inbounds begin
x = view(u,1:7) # x
y = view(u,8:14) # y
v = view(u,15:21) # x′
w = view(u,22:28) # y′
du[1:7] .= v
du[8:14].= w
for i in 15:28
du[i] = zero(u[1])
end
for i=1:7,j=1:7
if i != j
r = ((x[i]-x[j])^2 + (y[i] - y[j])^2)^(3/2)
du[14+i] += j*(x[j] - x[i])/r
du[21+i] += j*(y[j] - y[i])/r
end
end
end
end
# DynamicalOdeProblem
function dotv(dv,q,v,par,t)
@inbounds begin
x = view(q,1:7) # x
y = view(q,8:14) # y
vx = view(v,1:7) # x′
vy = view(v,8:14) # y′
for i in 1:14
dv[i] = zero(x[1])
end
for i=1:7,j=1:7
if i != j
r = ((x[i]-x[j])^2 + (y[i] - y[j])^2)^(3/2)
dv[i] += j*(x[j] - x[i])/r
dv[7+i] += j*(y[j] - y[i])/r
end
end
end
end
function dotq(dq,q,v,par,t)
@inbounds begin
x = view(q,1:7) # x
y = view(q,8:14) # y
vx = view(v,1:7) # x′
vy = view(v,8:14) # y′
dq[1:7] .= vx
dq[8:14].= vy
end
end
#
# Second Order Problem
#
function f2nd!(ddu,du,u,p,t)
@inbounds begin
x = view(u,1:7) # x
y = view(u,8:14) # y
vx = view(du,1:7) # x′
vy = view(du,8:14) # y′
for i in 1:14
ddu[i] = zero(x[1])
end
for i=1:7,j=1:7
if i != j
r = ((x[i]-x[j])^2 + (y[i] - y[j])^2)^(3/2)
ddu[i] += j*(x[j] - x[i])/r
ddu[7+i] += j*(y[j] - y[i])/r
end
end
end
end
| [
198,
2,
6682,
198,
198,
8818,
399,
2618,
28925,
7,
84,
11,
38,
76,
8,
198,
37811,
198,
220,
220,
220,
220,
399,
2618,
1917,
11582,
666,
357,
43476,
35610,
22819,
17540,
8,
198,
37811,
628,
220,
220,
220,
5391,
28,
17,
198,
220,
220,
220,
299,
2618,
28,
13664,
7,
38,
76,
8,
628,
2488,
259,
65,
3733,
2221,
198,
220,
220,
220,
2124,
796,
1570,
7,
84,
11,
16,
25,
22,
8,
220,
220,
1303,
2124,
198,
220,
220,
220,
331,
796,
1570,
7,
84,
11,
23,
25,
1415,
8,
220,
1303,
331,
198,
220,
220,
220,
410,
796,
1570,
7,
84,
11,
1314,
25,
2481,
8,
1303,
2124,
17478,
198,
220,
220,
220,
266,
796,
1570,
7,
84,
11,
1828,
25,
2078,
8,
1303,
331,
17478,
628,
220,
220,
220,
367,
28,
22570,
7,
417,
4906,
7,
84,
4008,
198,
220,
220,
220,
350,
28,
22570,
7,
417,
4906,
7,
84,
4008,
628,
220,
220,
220,
329,
1312,
287,
352,
25,
77,
2618,
198,
220,
220,
220,
220,
220,
220,
220,
367,
47932,
38,
76,
58,
72,
60,
9,
7,
85,
58,
72,
60,
9,
85,
58,
72,
48688,
86,
58,
72,
60,
9,
86,
58,
72,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
329,
474,
287,
1312,
10,
16,
25,
77,
2618,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
374,
796,
14808,
87,
58,
72,
45297,
87,
58,
73,
12962,
61,
17,
33747,
88,
58,
72,
45297,
88,
58,
73,
12962,
61,
17,
8,
61,
7,
16,
14,
17,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
350,
10,
16193,
38,
76,
58,
72,
60,
14,
81,
27493,
38,
76,
58,
73,
60,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1441,
7,
39,
14,
17,
12,
47,
8,
198,
220,
220,
220,
886,
198,
198,
437,
628,
198,
2,
440,
2934,
40781,
628,
198,
8818,
277,
7,
646,
11,
84,
11,
79,
11,
83,
8,
198,
220,
2488,
259,
65,
3733,
2221,
198,
220,
2124,
796,
1570,
7,
84,
11,
16,
25,
22,
8,
220,
220,
1303,
2124,
198,
220,
331,
796,
1570,
7,
84,
11,
23,
25,
1415,
8,
220,
1303,
331,
198,
220,
410,
796,
1570,
7,
84,
11,
1314,
25,
2481,
8,
1303,
2124,
17478,
198,
220,
266,
796,
1570,
7,
84,
11,
1828,
25,
2078,
8,
1303,
331,
17478,
198,
220,
7043,
58,
16,
25,
22,
60,
764,
28,
410,
198,
220,
7043,
58,
23,
25,
1415,
4083,
28,
266,
198,
220,
329,
1312,
287,
1315,
25,
2078,
198,
220,
220,
220,
7043,
58,
72,
60,
796,
6632,
7,
84,
58,
16,
12962,
198,
220,
886,
198,
220,
329,
1312,
28,
16,
25,
22,
11,
73,
28,
16,
25,
22,
198,
220,
220,
220,
611,
1312,
14512,
474,
198,
220,
220,
220,
220,
220,
374,
796,
14808,
87,
58,
72,
45297,
87,
58,
73,
12962,
61,
17,
1343,
357,
88,
58,
72,
60,
532,
331,
58,
73,
12962,
61,
17,
8,
61,
7,
18,
14,
17,
8,
198,
220,
220,
220,
220,
220,
7043,
58,
1415,
10,
72,
60,
15853,
474,
9,
7,
87,
58,
73,
60,
532,
2124,
58,
72,
12962,
14,
81,
198,
220,
220,
220,
220,
220,
7043,
58,
2481,
10,
72,
60,
15853,
474,
9,
7,
88,
58,
73,
60,
532,
331,
58,
72,
12962,
14,
81,
198,
220,
220,
220,
886,
198,
220,
886,
198,
220,
886,
198,
437,
628,
198,
2,
14970,
605,
46,
2934,
40781,
628,
198,
8818,
16605,
85,
7,
67,
85,
11,
80,
11,
85,
11,
1845,
11,
83,
8,
198,
31,
259,
65,
3733,
2221,
198,
220,
2124,
796,
1570,
7,
80,
11,
16,
25,
22,
8,
220,
220,
1303,
2124,
198,
220,
331,
796,
1570,
7,
80,
11,
23,
25,
1415,
8,
220,
1303,
331,
198,
220,
410,
87,
796,
1570,
7,
85,
11,
16,
25,
22,
8,
220,
220,
1303,
2124,
17478,
198,
220,
410,
88,
796,
1570,
7,
85,
11,
23,
25,
1415,
8,
220,
1303,
331,
17478,
198,
220,
329,
1312,
287,
352,
25,
1415,
198,
220,
220,
220,
288,
85,
58,
72,
60,
796,
6632,
7,
87,
58,
16,
12962,
198,
220,
886,
198,
220,
329,
1312,
28,
16,
25,
22,
11,
73,
28,
16,
25,
22,
198,
220,
220,
220,
611,
1312,
14512,
474,
198,
220,
220,
220,
220,
220,
374,
796,
14808,
87,
58,
72,
45297,
87,
58,
73,
12962,
61,
17,
1343,
357,
88,
58,
72,
60,
532,
331,
58,
73,
12962,
61,
17,
8,
61,
7,
18,
14,
17,
8,
198,
220,
220,
220,
220,
220,
288,
85,
58,
72,
60,
15853,
474,
9,
7,
87,
58,
73,
60,
532,
2124,
58,
72,
12962,
14,
81,
198,
220,
220,
220,
220,
220,
288,
85,
58,
22,
10,
72,
60,
15853,
474,
9,
7,
88,
58,
73,
60,
532,
331,
58,
72,
12962,
14,
81,
198,
220,
220,
220,
886,
198,
220,
886,
198,
220,
886,
198,
437,
628,
198,
8818,
16605,
80,
7,
49506,
11,
80,
11,
85,
11,
1845,
11,
83,
8,
198,
31,
259,
65,
3733,
2221,
198,
220,
2124,
796,
1570,
7,
80,
11,
16,
25,
22,
8,
220,
220,
1303,
2124,
198,
220,
331,
796,
1570,
7,
80,
11,
23,
25,
1415,
8,
220,
1303,
331,
198,
220,
410,
87,
796,
1570,
7,
85,
11,
16,
25,
22,
8,
220,
220,
1303,
2124,
17478,
198,
220,
410,
88,
796,
1570,
7,
85,
11,
23,
25,
1415,
8,
220,
1303,
331,
17478,
198,
220,
288,
80,
58,
16,
25,
22,
60,
764,
28,
410,
87,
198,
220,
288,
80,
58,
23,
25,
1415,
4083,
28,
410,
88,
198,
220,
886,
198,
437,
628,
198,
2,
198,
2,
220,
5498,
8284,
20647,
198,
2,
198,
198,
8818,
277,
17,
358,
0,
7,
67,
646,
11,
646,
11,
84,
11,
79,
11,
83,
8,
198,
220,
2488,
259,
65,
3733,
2221,
198,
220,
2124,
796,
1570,
7,
84,
11,
16,
25,
22,
8,
220,
220,
1303,
2124,
198,
220,
331,
796,
1570,
7,
84,
11,
23,
25,
1415,
8,
220,
1303,
331,
198,
220,
410,
87,
796,
1570,
7,
646,
11,
16,
25,
22,
8,
1303,
2124,
17478,
198,
220,
410,
88,
796,
1570,
7,
646,
11,
23,
25,
1415,
8,
1303,
331,
17478,
628,
220,
329,
1312,
287,
352,
25,
1415,
198,
220,
220,
220,
288,
646,
58,
72,
60,
796,
6632,
7,
87,
58,
16,
12962,
198,
220,
886,
628,
220,
329,
1312,
28,
16,
25,
22,
11,
73,
28,
16,
25,
22,
198,
220,
220,
220,
611,
1312,
14512,
474,
198,
220,
220,
220,
220,
220,
374,
796,
14808,
87,
58,
72,
45297,
87,
58,
73,
12962,
61,
17,
1343,
357,
88,
58,
72,
60,
532,
331,
58,
73,
12962,
61,
17,
8,
61,
7,
18,
14,
17,
8,
198,
220,
220,
220,
220,
220,
288,
646,
58,
72,
60,
15853,
474,
9,
7,
87,
58,
73,
60,
532,
2124,
58,
72,
12962,
14,
81,
198,
220,
220,
220,
220,
220,
288,
646,
58,
22,
10,
72,
60,
15853,
474,
9,
7,
88,
58,
73,
60,
532,
331,
58,
72,
12962,
14,
81,
198,
220,
220,
220,
886,
198,
220,
886,
198,
220,
886,
198,
437,
198
] | 1.578035 | 1,211 |
f (1)
| [
69,
357,
16,
8,
198
] | 1.2 | 5 |
# Histogram (Bar) plots
# Vertical bars
function PlotBars(label_id, x::AbstractArray{T1}, y::AbstractArray{T2},
args...) where {T1<:Real,T2<:Real}
return PlotBars(label_id, promote(x, y)..., args...)
end
function PlotBars(values::AbstractArray{T}; count::Integer=length(values),
label_id::String="", width=0.67, shift=0.0, offset::Integer=0,
stride::Integer=1) where {T<:ImPlotData}
return PlotBars(label_id, values, count, width, shift, offset, stride * sizeof(T))
end
function PlotBars(x::AbstractArray{T}, y::AbstractArray{T};
count::Integer=min(length(x), length(y)), label_id::String="", width=0.67,
offset::Integer=0, stride::Integer=1) where {T<:ImPlotData}
return PlotBars(label_id, x, y, count, width, offset, stride * sizeof(T))
end
function PlotBars(x::AbstractArray{T1}, y::AbstractArray{T2};
kwargs...) where {T1<:Real,T2<:Real}
return PlotBars(promote(x, y)..., kwargs...)
end
# Horizontal bars
function PlotBarsH(label_id, x::AbstractArray{T1}, y::AbstractArray{T2},
args...) where {T1<:Real,T2<:Real}
return PlotBarsH(label_id, promote(x, y)..., args...)
end
function PlotBarsH(values::AbstractArray{T}; count::Integer=length(values),
label_id::String="", width=0.67, shift=0.0, offset::Integer=0,
stride::Integer=1) where {T<:ImPlotData}
return PlotBarsH(label_id, values, count, width, shift, offset, stride * sizeof(T))
end
function PlotBarsH(x::AbstractArray{T}, y::AbstractArray{T};
count::Integer=min(length(x), length(y)), label_id::String="",
width=0.67, offset::Integer=0, stride::Integer=1) where {T<:ImPlotData}
return PlotBarsH(label_id, x, y, count, width, offset, stride * sizeof(T))
end
function PlotBarsH(x::AbstractArray{T1}, y::AbstractArray{T2};
kwargs...) where {T1<:Real,T2<:Real}
return PlotBarsH(promote(x, y)..., kwargs...)
end
| [
2,
5590,
21857,
357,
10374,
8,
21528,
198,
2,
38937,
9210,
198,
8818,
28114,
33,
945,
7,
18242,
62,
312,
11,
2124,
3712,
23839,
19182,
90,
51,
16,
5512,
331,
3712,
23839,
19182,
90,
51,
17,
5512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
26498,
23029,
810,
1391,
51,
16,
27,
25,
15633,
11,
51,
17,
27,
25,
15633,
92,
198,
220,
220,
220,
1441,
28114,
33,
945,
7,
18242,
62,
312,
11,
7719,
7,
87,
11,
331,
26513,
11,
26498,
23029,
198,
437,
198,
198,
8818,
28114,
33,
945,
7,
27160,
3712,
23839,
19182,
90,
51,
19629,
954,
3712,
46541,
28,
13664,
7,
27160,
828,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6167,
62,
312,
3712,
10100,
2625,
1600,
9647,
28,
15,
13,
3134,
11,
6482,
28,
15,
13,
15,
11,
11677,
3712,
46541,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
33769,
3712,
46541,
28,
16,
8,
810,
1391,
51,
27,
25,
3546,
43328,
6601,
92,
198,
220,
220,
220,
1441,
28114,
33,
945,
7,
18242,
62,
312,
11,
3815,
11,
954,
11,
9647,
11,
6482,
11,
11677,
11,
33769,
1635,
39364,
7,
51,
4008,
198,
437,
198,
198,
8818,
28114,
33,
945,
7,
87,
3712,
23839,
19182,
90,
51,
5512,
331,
3712,
23839,
19182,
90,
51,
19629,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
954,
3712,
46541,
28,
1084,
7,
13664,
7,
87,
828,
4129,
7,
88,
36911,
6167,
62,
312,
3712,
10100,
2625,
1600,
9647,
28,
15,
13,
3134,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
11677,
3712,
46541,
28,
15,
11,
33769,
3712,
46541,
28,
16,
8,
810,
1391,
51,
27,
25,
3546,
43328,
6601,
92,
198,
220,
220,
220,
1441,
28114,
33,
945,
7,
18242,
62,
312,
11,
2124,
11,
331,
11,
954,
11,
9647,
11,
11677,
11,
33769,
1635,
39364,
7,
51,
4008,
198,
437,
198,
198,
8818,
28114,
33,
945,
7,
87,
3712,
23839,
19182,
90,
51,
16,
5512,
331,
3712,
23839,
19182,
90,
51,
17,
19629,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
479,
86,
22046,
23029,
810,
1391,
51,
16,
27,
25,
15633,
11,
51,
17,
27,
25,
15633,
92,
198,
220,
220,
220,
1441,
28114,
33,
945,
7,
16963,
1258,
7,
87,
11,
331,
26513,
11,
479,
86,
22046,
23029,
198,
437,
198,
198,
2,
6075,
38342,
9210,
198,
8818,
28114,
33,
945,
39,
7,
18242,
62,
312,
11,
2124,
3712,
23839,
19182,
90,
51,
16,
5512,
331,
3712,
23839,
19182,
90,
51,
17,
5512,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
26498,
23029,
810,
1391,
51,
16,
27,
25,
15633,
11,
51,
17,
27,
25,
15633,
92,
198,
220,
220,
220,
1441,
28114,
33,
945,
39,
7,
18242,
62,
312,
11,
7719,
7,
87,
11,
331,
26513,
11,
26498,
23029,
198,
437,
198,
198,
8818,
28114,
33,
945,
39,
7,
27160,
3712,
23839,
19182,
90,
51,
19629,
954,
3712,
46541,
28,
13664,
7,
27160,
828,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
6167,
62,
312,
3712,
10100,
2625,
1600,
9647,
28,
15,
13,
3134,
11,
6482,
28,
15,
13,
15,
11,
11677,
3712,
46541,
28,
15,
11,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
33769,
3712,
46541,
28,
16,
8,
810,
1391,
51,
27,
25,
3546,
43328,
6601,
92,
198,
220,
220,
220,
1441,
28114,
33,
945,
39,
7,
18242,
62,
312,
11,
3815,
11,
954,
11,
9647,
11,
6482,
11,
11677,
11,
33769,
1635,
39364,
7,
51,
4008,
198,
437,
198,
198,
8818,
28114,
33,
945,
39,
7,
87,
3712,
23839,
19182,
90,
51,
5512,
331,
3712,
23839,
19182,
90,
51,
19629,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
954,
3712,
46541,
28,
1084,
7,
13664,
7,
87,
828,
4129,
7,
88,
36911,
6167,
62,
312,
3712,
10100,
2625,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
9647,
28,
15,
13,
3134,
11,
11677,
3712,
46541,
28,
15,
11,
33769,
3712,
46541,
28,
16,
8,
810,
1391,
51,
27,
25,
3546,
43328,
6601,
92,
198,
220,
220,
220,
1441,
28114,
33,
945,
39,
7,
18242,
62,
312,
11,
2124,
11,
331,
11,
954,
11,
9647,
11,
11677,
11,
33769,
1635,
39364,
7,
51,
4008,
198,
437,
198,
198,
8818,
28114,
33,
945,
39,
7,
87,
3712,
23839,
19182,
90,
51,
16,
5512,
331,
3712,
23839,
19182,
90,
51,
17,
19629,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
479,
86,
22046,
23029,
810,
1391,
51,
16,
27,
25,
15633,
11,
51,
17,
27,
25,
15633,
92,
198,
220,
220,
220,
1441,
28114,
33,
945,
39,
7,
16963,
1258,
7,
87,
11,
331,
26513,
11,
479,
86,
22046,
23029,
198,
437,
198
] | 2.269575 | 894 |
using RecipesBase
export SeqPlot
export TestPlot
export rectangle_corners
function rectangle_corners(x::Real,y::Real,w,h; anchor=:bottomright)
if anchor == :botttomright
[x,x+w,x+w,x], [y,y,y+h,y+h]
elseif anchor == :center
[x-w/2, x+w/2, x+w/2, x-w/2], [y-h/2, y-h/2, y+h/2, y+h/2]
else
error("Anchor not recognised.")
end
end
function rectangle_corners(
x_vec::Vector{T},y_vec::Vector{T},
w,h; anchor=:bottomleft
) where {T<:Real}
x_out = Vector{Real}[]
y_out = Vector{Real}[]
if anchor == :bottomleft
for (x,y) in zip(x_vec, y_vec)
push!(x_out, [x,x+w,x+w,x])
push!(y_out, [y,y,y+h,y+h])
end
elseif anchor == :center
for (x,y) in zip(x_vec, y_vec)
push!(x_out, [x-w/2, x+w/2, x+w/2, x-w/2])
push!(y_out, [y-h/2, y-h/2, y+h/2, y+h/2])
end
else
error("Anchor not recognised.")
end
x_out, y_out
end
@userplot SeqPlot
@recipe function f(
h::SeqPlot;
entrymargin=0.1,
entryfontsize=20
)
seq = h.args[1]
w,h = (1,1)
y = fill(0.0, length(seq))
x = [0.0 + i*(w+entrymargin) for i in 0:(length(seq)-1)]
annotations := [(x,y,string(i)) for (x,y,i) in zip(x,y,seq)]
annotationfontsize := entryfontsize
x_cords, y_cords = rectangle_corners(x,y, w, h; anchor=:center)
showaxis --> false
axis --> nothing
aspect_ratio --> 1
legend --> false
@series begin
seriestype := :shape
x_cords,y_cords
end
end
@userplot TestPlot
@recipe function f(
h::TestPlot
)
aspect_ratio --> 1
showaxis --> false
@series begin
seriestype := :shape
1:4,1:4
end
@series begin
seriestype := :line
1:4, 1:4
annotations := [[];[(i,i,string(i)) for i in 1:4]]
annotationhalign --> :right
end
end | [
3500,
44229,
14881,
198,
39344,
1001,
80,
43328,
198,
39344,
6208,
43328,
198,
39344,
35991,
62,
20772,
364,
198,
198,
8818,
35991,
62,
20772,
364,
7,
87,
3712,
15633,
11,
88,
3712,
15633,
11,
86,
11,
71,
26,
18021,
28,
25,
22487,
3506,
8,
198,
220,
220,
220,
611,
18021,
6624,
1058,
13645,
926,
296,
3506,
220,
198,
220,
220,
220,
220,
220,
220,
220,
685,
87,
11,
87,
10,
86,
11,
87,
10,
86,
11,
87,
4357,
685,
88,
11,
88,
11,
88,
10,
71,
11,
88,
10,
71,
60,
198,
220,
220,
220,
2073,
361,
18021,
6624,
1058,
16159,
220,
198,
220,
220,
220,
220,
220,
220,
220,
685,
87,
12,
86,
14,
17,
11,
2124,
10,
86,
14,
17,
11,
2124,
10,
86,
14,
17,
11,
2124,
12,
86,
14,
17,
4357,
685,
88,
12,
71,
14,
17,
11,
331,
12,
71,
14,
17,
11,
331,
10,
71,
14,
17,
11,
331,
10,
71,
14,
17,
60,
198,
220,
220,
220,
2073,
220,
198,
220,
220,
220,
220,
220,
220,
220,
4049,
7203,
2025,
354,
273,
407,
20915,
19570,
198,
220,
220,
220,
886,
220,
198,
437,
220,
198,
198,
8818,
35991,
62,
20772,
364,
7,
198,
220,
220,
220,
2124,
62,
35138,
3712,
38469,
90,
51,
5512,
88,
62,
35138,
3712,
38469,
90,
51,
5512,
198,
220,
220,
220,
266,
11,
71,
26,
18021,
28,
25,
22487,
9464,
198,
220,
220,
220,
1267,
810,
1391,
51,
27,
25,
15633,
92,
628,
220,
220,
220,
2124,
62,
448,
796,
20650,
90,
15633,
92,
21737,
198,
220,
220,
220,
331,
62,
448,
796,
20650,
90,
15633,
92,
21737,
198,
220,
220,
220,
611,
18021,
6624,
1058,
22487,
9464,
198,
220,
220,
220,
220,
220,
220,
220,
329,
357,
87,
11,
88,
8,
287,
19974,
7,
87,
62,
35138,
11,
331,
62,
35138,
8,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
87,
62,
448,
11,
685,
87,
11,
87,
10,
86,
11,
87,
10,
86,
11,
87,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
88,
62,
448,
11,
685,
88,
11,
88,
11,
88,
10,
71,
11,
88,
10,
71,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
2073,
361,
18021,
6624,
1058,
16159,
220,
198,
220,
220,
220,
220,
220,
220,
220,
329,
357,
87,
11,
88,
8,
287,
19974,
7,
87,
62,
35138,
11,
331,
62,
35138,
8,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
87,
62,
448,
11,
685,
87,
12,
86,
14,
17,
11,
2124,
10,
86,
14,
17,
11,
2124,
10,
86,
14,
17,
11,
2124,
12,
86,
14,
17,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
88,
62,
448,
11,
685,
88,
12,
71,
14,
17,
11,
331,
12,
71,
14,
17,
11,
331,
10,
71,
14,
17,
11,
331,
10,
71,
14,
17,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
2073,
220,
198,
220,
220,
220,
220,
220,
220,
220,
4049,
7203,
2025,
354,
273,
407,
20915,
19570,
198,
220,
220,
220,
886,
220,
198,
220,
220,
220,
2124,
62,
448,
11,
331,
62,
448,
198,
437,
220,
198,
198,
31,
7220,
29487,
1001,
80,
43328,
198,
31,
29102,
431,
2163,
277,
7,
198,
220,
220,
220,
289,
3712,
4653,
80,
43328,
26,
220,
198,
220,
220,
220,
5726,
36153,
28,
15,
13,
16,
11,
220,
198,
220,
220,
220,
5726,
10331,
7857,
28,
1238,
198,
220,
220,
220,
1267,
198,
220,
220,
220,
33756,
796,
289,
13,
22046,
58,
16,
60,
198,
220,
220,
220,
266,
11,
71,
796,
357,
16,
11,
16,
8,
220,
198,
220,
220,
220,
331,
796,
6070,
7,
15,
13,
15,
11,
4129,
7,
41068,
4008,
198,
220,
220,
220,
2124,
796,
685,
15,
13,
15,
1343,
1312,
9,
7,
86,
10,
13000,
36153,
8,
329,
1312,
287,
657,
37498,
13664,
7,
41068,
13219,
16,
15437,
198,
220,
220,
220,
37647,
19039,
47527,
87,
11,
88,
11,
8841,
7,
72,
4008,
329,
357,
87,
11,
88,
11,
72,
8,
287,
19974,
7,
87,
11,
88,
11,
41068,
15437,
198,
220,
220,
220,
23025,
10331,
7857,
19039,
5726,
10331,
7857,
198,
220,
220,
220,
2124,
62,
66,
3669,
11,
331,
62,
66,
3669,
796,
35991,
62,
20772,
364,
7,
87,
11,
88,
11,
266,
11,
289,
26,
18021,
28,
25,
16159,
8,
198,
220,
220,
220,
905,
22704,
14610,
3991,
220,
198,
220,
220,
220,
16488,
14610,
2147,
220,
198,
220,
220,
220,
4843,
62,
10366,
952,
14610,
352,
220,
198,
220,
220,
220,
8177,
14610,
3991,
220,
198,
220,
220,
220,
2488,
25076,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
1055,
6386,
2981,
19039,
1058,
43358,
198,
220,
220,
220,
220,
220,
220,
220,
2124,
62,
66,
3669,
11,
88,
62,
66,
3669,
198,
220,
220,
220,
886,
220,
198,
437,
220,
198,
198,
31,
7220,
29487,
6208,
43328,
198,
31,
29102,
431,
2163,
277,
7,
198,
220,
220,
220,
289,
3712,
14402,
43328,
198,
220,
220,
220,
1267,
198,
220,
220,
220,
4843,
62,
10366,
952,
14610,
352,
198,
220,
220,
220,
905,
22704,
14610,
3991,
220,
198,
220,
220,
220,
2488,
25076,
2221,
220,
198,
220,
220,
220,
220,
220,
220,
220,
1055,
6386,
2981,
19039,
1058,
43358,
198,
220,
220,
220,
220,
220,
220,
220,
352,
25,
19,
11,
16,
25,
19,
198,
220,
220,
220,
886,
220,
198,
220,
220,
220,
2488,
25076,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
1055,
6386,
2981,
19039,
1058,
1370,
198,
220,
220,
220,
220,
220,
220,
220,
352,
25,
19,
11,
352,
25,
19,
198,
220,
220,
220,
220,
220,
220,
220,
37647,
19039,
16410,
11208,
58,
7,
72,
11,
72,
11,
8841,
7,
72,
4008,
329,
1312,
287,
352,
25,
19,
11907,
198,
220,
220,
220,
220,
220,
220,
220,
23025,
14201,
570,
14610,
1058,
3506,
198,
220,
220,
220,
886,
198,
437,
220
] | 1.892399 | 1,013 |
## Exercise 4-8
## Enter the code in this chapter in a notebook.
## 1. Draw a stack diagram that shows the state of the program while executing circle(🐢, radius).
## You can do the arithmetic by hand or add print statements to the code.
println("Ans 1: ")
println(" turtle --> Turtle")
println(" turtle --> Turtle")
println(" radius --> 100")
println(" circumference --> 628.318...")
println(" n --> 212")
println(" len --> 2")
println(" turtle --> Turtle")
println(" nsides --> 212")
println(" len --> 2")
println(" i --> 1:212")
println(" return")
## 2. The version of arc in Refactoring is not very accurate because the linear approximation of the circle
## is always outside the true circle. As a result, the turtle ends up a few pixels away from the correct destination.
## My solution shows a way to reduce the effect of this error. Read the code and see if it makes sense to you.
## If you draw a diagram, you might see how it works.
using ThinkJulia
function polyline(t, n, len, angle)
for i in 1:n
forward(t, len)
turn(t, -angle)
end
end
"""
arc(t, r, angle)
Draws an arc with the given radius and angle:
t: turtle
r: radius
angle: angle subtended by the arc, in degrees
"""
function arc(t, r, angle)
arc_len = 2 * π * r * abs(angle) / 360
n = trunc(arc_len / 4) + 3
step_len = arc_len / n
step_angle = angle / n
# making a slight left turn before starting reduces
# the error caused by the linear approximation of the arc
turn(t, -step_angle/2)
polyline(t, n, step_len, step_angle)
turn(t, step_angle/2)
end
println("Ans 2: ")
@svg begin
turtle = Turtle()
# forward(turtle, 30) # for checking the position of the turtle
arc(turtle, 100, 125)
end
##
println("End.")
| [
2235,
32900,
604,
12,
23,
198,
2235,
6062,
262,
2438,
287,
428,
6843,
287,
257,
20922,
13,
198,
198,
2235,
352,
13,
15315,
257,
8931,
16362,
326,
2523,
262,
1181,
286,
262,
1430,
981,
23710,
9197,
7,
8582,
238,
95,
11,
16874,
737,
220,
198,
2235,
921,
460,
466,
262,
34768,
416,
1021,
393,
751,
3601,
6299,
284,
262,
2438,
13,
198,
35235,
7203,
2025,
82,
352,
25,
366,
8,
198,
198,
35235,
7203,
28699,
14610,
33137,
4943,
198,
35235,
7203,
220,
220,
220,
220,
28699,
14610,
33137,
4943,
198,
35235,
7203,
220,
220,
220,
220,
16874,
14610,
1802,
4943,
198,
35235,
7203,
220,
220,
220,
220,
38447,
14610,
718,
2078,
13,
36042,
9313,
8,
198,
35235,
7203,
220,
220,
220,
220,
299,
14610,
23679,
4943,
198,
35235,
7203,
220,
220,
220,
220,
18896,
14610,
362,
4943,
198,
35235,
7203,
220,
220,
220,
220,
220,
220,
220,
220,
28699,
14610,
33137,
4943,
198,
35235,
7203,
220,
220,
220,
220,
220,
220,
220,
220,
36545,
1460,
14610,
23679,
4943,
198,
35235,
7203,
220,
220,
220,
220,
220,
220,
220,
220,
18896,
14610,
362,
4943,
198,
35235,
7203,
220,
220,
220,
220,
220,
220,
220,
220,
1312,
14610,
352,
25,
21777,
4943,
198,
35235,
7203,
1441,
4943,
198,
198,
2235,
362,
13,
383,
2196,
286,
10389,
287,
6524,
529,
3255,
318,
407,
845,
7187,
780,
262,
14174,
40874,
286,
262,
9197,
220,
198,
2235,
318,
1464,
2354,
262,
2081,
9197,
13,
1081,
257,
1255,
11,
262,
28699,
5645,
510,
257,
1178,
17848,
1497,
422,
262,
3376,
10965,
13,
220,
198,
2235,
2011,
4610,
2523,
257,
835,
284,
4646,
262,
1245,
286,
428,
4049,
13,
4149,
262,
2438,
290,
766,
611,
340,
1838,
2565,
284,
345,
13,
220,
198,
2235,
1002,
345,
3197,
257,
16362,
11,
345,
1244,
766,
703,
340,
2499,
13,
198,
3500,
11382,
16980,
544,
198,
198,
8818,
7514,
1370,
7,
83,
11,
299,
11,
18896,
11,
9848,
8,
198,
220,
220,
220,
329,
1312,
287,
352,
25,
77,
198,
220,
220,
220,
220,
220,
220,
220,
2651,
7,
83,
11,
18896,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1210,
7,
83,
11,
532,
9248,
8,
198,
220,
220,
220,
886,
198,
437,
198,
198,
37811,
198,
5605,
7,
83,
11,
374,
11,
9848,
8,
198,
198,
25302,
82,
281,
10389,
351,
262,
1813,
16874,
290,
9848,
25,
628,
220,
220,
220,
256,
25,
28699,
198,
220,
220,
220,
374,
25,
16874,
198,
220,
220,
220,
9848,
25,
9848,
13284,
1631,
416,
262,
10389,
11,
287,
7370,
198,
37811,
198,
8818,
10389,
7,
83,
11,
374,
11,
9848,
8,
198,
220,
220,
220,
10389,
62,
11925,
796,
362,
1635,
18074,
222,
1635,
374,
1635,
2352,
7,
9248,
8,
1220,
11470,
198,
220,
220,
220,
299,
796,
40122,
7,
5605,
62,
11925,
1220,
604,
8,
1343,
513,
198,
220,
220,
220,
2239,
62,
11925,
796,
10389,
62,
11925,
1220,
299,
198,
220,
220,
220,
2239,
62,
9248,
796,
9848,
1220,
299,
628,
220,
220,
220,
1303,
1642,
257,
3731,
1364,
1210,
878,
3599,
12850,
198,
220,
220,
220,
1303,
262,
4049,
4073,
416,
262,
14174,
40874,
286,
262,
10389,
198,
220,
220,
220,
1210,
7,
83,
11,
532,
9662,
62,
9248,
14,
17,
8,
198,
220,
220,
220,
7514,
1370,
7,
83,
11,
299,
11,
2239,
62,
11925,
11,
2239,
62,
9248,
8,
198,
220,
220,
220,
1210,
7,
83,
11,
2239,
62,
9248,
14,
17,
8,
198,
437,
198,
198,
35235,
7203,
2025,
82,
362,
25,
366,
8,
198,
198,
31,
21370,
70,
2221,
198,
220,
220,
220,
28699,
796,
33137,
3419,
198,
220,
220,
220,
1303,
2651,
7,
83,
17964,
11,
1542,
8,
220,
1303,
329,
10627,
262,
2292,
286,
262,
28699,
198,
220,
220,
220,
10389,
7,
83,
17964,
11,
1802,
11,
13151,
8,
198,
437,
198,
198,
2235,
220,
198,
198,
35235,
7203,
12915,
19570,
198
] | 2.853354 | 641 |
include("ctqw/ctqw.jl")
include("szegedy/szegedy.jl")
| [
17256,
7203,
310,
80,
86,
14,
310,
80,
86,
13,
20362,
4943,
198,
17256,
7203,
82,
89,
1533,
4716,
14,
82,
89,
1533,
4716,
13,
20362,
4943,
198
] | 1.928571 | 28 |
##########
##########
# A check of the distribution of shapes of biomass and richness
# in relevant paper
##########
##########
# AUTHOR: Cole B. Brookson
# DATE OF CREATION: 2021-05-17
##########
##########
# set up =======================================================================
using DrWatson
@quickactivate "trait-based-rewiring"
# set packages
using DataFrames, Plots, StatsPlots
include(srcdir("01_eqn_component_functions.jl"))
# set all positional values
organism_id_pos = 1
organism_type_pos = 2
body_size_pos = 3
lower_prey_limit_pos = 4
upper_prey_limit_pos = 5
habitat_midpoint_pos = 6
habitat_lower_limit_pos = 7
habitat_upper_limit_pos = 8
nutritional_value_pos = 9
biomass_pos = 10
network_id_pos = 11
alive_pos = 12
# perform loop to grab all values ==============================================
# draw 100 networks of the biomass/richness shapes and look at how they group
richness_biomass_comp = Array{Any}(undef, (450000, 5))
j = 1
for i in 1:1000
for richness in ["top-heavy", "bottom-heavy", "uniform"]
for biomass in ["pyramid", "uniform", "inverted"]
temp = Array{Any}(undef, (50, 5))
species_list = network_draw(50, richness)[1]
species_list = initialize_biomass(species_list, biomass)
for k in 1:size(species_list, 1)
temp[k, 1] = richness
temp[k, 2] = biomass
temp[k, 3] = species_list[k, body_size_pos]
temp[k, 4] = species_list[k, habitat_midpoint_pos]
temp[k, 5] = species_list[k, organism_type_pos]
end
richness_biomass_comp[(j:j+49), :] = temp
j = j + 50
end
end
end
# turn array into dataframe
richness_biomass_comp = DataFrame(
richness_biomass_comp,
[:richness_shape, :biomass_shape, :body_size, :biomass, :org_type]
)
# make plots of the 9 combos ===================================================
# top-heavy & pyramid
topheavy_pryamid_data = DataFrame(filter(
(row -> row.richness_shape == "top-heavy" &&
row.biomass_shape == "pyramid"),
richness_biomass_comp
))
topheavy_pryamid_plot = @df topheavy_pryamid_data plot(
:body_size,
:biomass,
group = :org_type,
seriestype = :scatter,
xlabel = "body size",
ylabel = "biomass",
legend = false
#title = "Richness = top-heavy, biomass = pyramid"
)
# top-heavy & uniform
topheavy_uniform_data = filter(
(row -> row.richness_shape == "top-heavy" &&
row.biomass_shape == "uniform"),
richness_biomass_comp
)
topheavy_uniform_plot = @df topheavy_uniform_data plot(
:body_size,
:biomass,
group = :org_type,
seriestype = :scatter,
xlabel = "body size",
ylabel = "biomass",
legend = false
#title = "Richness = top-heavy, biomass = uniform"
)
# top-heavy and inverted
topheavy_inverted_data = filter(
(row -> row.richness_shape == "top-heavy" &&
row.biomass_shape == "inverted"),
richness_biomass_comp
)
topheavy_inverted_plot = @df topheavy_inverted_data plot(
:body_size,
:biomass,
group = :org_type,
seriestype = :scatter,
xlabel = "body size",
ylabel = "biomass",
legend = false
#title = "Richness = top-heavy, biomass = inverted"
)
# uniform and pyramid
uniform_pyramid_data = filter(
(row -> row.richness_shape == "uniform" &&
row.biomass_shape == "pyramid"),
richness_biomass_comp
)
uniform_pyramid_plot = @df uniform_pyramid_data plot(
:body_size,
:biomass,
group = :org_type,
seriestype = :scatter,
xlabel = "body size",
ylabel = "biomass",
legend = false
#title = "Richness = uniform, biomass = pyramid"
)
# uniform and uniform
uniform_uniform_data = filter(
(row -> row.richness_shape == "uniform" &&
row.biomass_shape == "uniform"),
richness_biomass_comp
)
uniform_uniform_plot = @df uniform_uniform_data plot(
:body_size,
:biomass,
group = :org_type,
seriestype = :scatter,
xlabel = "body size",
ylabel = "biomass",
legend = false
#title = "Richness = uniform, biomass = uniform"
)
# uniform and inverted
uniform_inverted_data = filter(
(row -> row.richness_shape == "uniform" &&
row.biomass_shape == "inverted"),
richness_biomass_comp
)
uniform_inverted_plot = @df uniform_inverted_data plot(
:body_size,
:biomass,
group = :org_type,
seriestype = :scatter,
xlabel = "body size",
ylabel = "biomass",
legend = false
#title = "Richness = uniform, biomass = inverted"
)
# bottom-heavy and pyramid
bottomheavy_pryamid_data = filter(
(row -> row.richness_shape == "bottom-heavy" &&
row.biomass_shape == "pyramid"),
richness_biomass_comp
)
bottomheavy_pryamid_plot = @df bottomheavy_pryamid_data plot(
:body_size,
:biomass,
group = :org_type,
seriestype = :scatter,
xlabel = "body size",
ylabel = "biomass",
legend = false
#title = "Richness = bottom-heavy, biomass = pyramid"
)
# bottom-heavy and uniform
bottomheavy_uniform_data = filter(
(row -> row.richness_shape == "bottom-heavy" &&
row.biomass_shape == "uniform"),
richness_biomass_comp
)
bottomheavy_uniform_plot = @df bottomheavy_uniform_data plot(
:body_size,
:biomass,
group = :org_type,
seriestype = :scatter,
xlabel = "body size",
ylabel = "biomass",
legend = false
#title = "Richness = bottom-heavy, biomass = uniform"
)
# bottom-heavy and inverted
bottomheavy_inverted_data = filter(
(row -> row.richness_shape == "bottom-heavy" &&
row.biomass_shape == "inverted"),
richness_biomass_comp
)
bottomheavy_inverted_plot = @df bottomheavy_inverted_data plot(
:body_size,
:biomass,
group = :org_type,
seriestype = :scatter,
xlabel = "body size",
ylabel = "biomass",
#title = "Richness = bottom-heavy, biomass = inverted",
legend = false
)
# put all plots together
all_shapes_plots = Plots.plot(
topheavy_pryamid_plot,
topheavy_uniform_plot,
topheavy_inverted_plot,
uniform_pyramid_plot,
uniform_uniform_plot,
uniform_inverted_plot,
bottomheavy_pryamid_plot,
bottomheavy_uniform_plot,
bottomheavy_inverted_plot,
layout = (3,3),
#legend = false,
#layout = Plots.grid(3,3, heights = [0.3, 0.3, 0.3]),
#xlabel = "body size",
#ylabel = "biomass"
title = ["1", "2", "3", "4", "5", "6", "7", "8", "9"]
)
Plots.savefig(all_shapes_plots, "./figs/all_shapes_plots.png")
| [
7804,
2235,
220,
198,
7804,
2235,
198,
2,
317,
2198,
286,
262,
6082,
286,
15268,
286,
42584,
290,
46792,
198,
2,
287,
5981,
3348,
198,
7804,
2235,
198,
7804,
2235,
198,
2,
44746,
25,
11768,
347,
13,
7590,
1559,
198,
2,
360,
6158,
3963,
29244,
6234,
25,
33448,
12,
2713,
12,
1558,
198,
7804,
2235,
198,
7804,
2235,
198,
198,
2,
900,
510,
38093,
50155,
198,
3500,
1583,
54,
13506,
198,
31,
24209,
39022,
366,
9535,
270,
12,
3106,
12,
1809,
3428,
1,
198,
198,
2,
900,
10392,
198,
3500,
6060,
35439,
11,
1345,
1747,
11,
20595,
3646,
1747,
198,
198,
17256,
7,
10677,
15908,
7203,
486,
62,
27363,
77,
62,
42895,
62,
12543,
2733,
13,
20362,
48774,
198,
198,
2,
900,
477,
45203,
3815,
220,
198,
9971,
1042,
62,
312,
62,
1930,
796,
352,
198,
9971,
1042,
62,
4906,
62,
1930,
796,
362,
198,
2618,
62,
7857,
62,
1930,
796,
513,
198,
21037,
62,
3866,
88,
62,
32374,
62,
1930,
796,
604,
198,
45828,
62,
3866,
88,
62,
32374,
62,
1930,
796,
642,
198,
5976,
270,
265,
62,
13602,
4122,
62,
1930,
796,
718,
198,
5976,
270,
265,
62,
21037,
62,
32374,
62,
1930,
796,
767,
198,
5976,
270,
265,
62,
45828,
62,
32374,
62,
1930,
796,
807,
198,
14930,
21297,
62,
8367,
62,
1930,
796,
860,
198,
8482,
296,
562,
62,
1930,
796,
838,
198,
27349,
62,
312,
62,
1930,
796,
1367,
198,
282,
425,
62,
1930,
796,
1105,
198,
198,
2,
1620,
9052,
284,
5552,
477,
3815,
46111,
25609,
28,
198,
198,
2,
3197,
1802,
7686,
286,
262,
42584,
14,
7527,
1108,
15268,
290,
804,
379,
703,
484,
1448,
220,
198,
7527,
1108,
62,
8482,
296,
562,
62,
5589,
796,
15690,
90,
7149,
92,
7,
917,
891,
11,
357,
2231,
2388,
11,
642,
4008,
198,
73,
796,
352,
198,
1640,
1312,
287,
352,
25,
12825,
198,
220,
220,
220,
329,
46792,
287,
14631,
4852,
12,
23701,
1600,
366,
22487,
12,
23701,
1600,
366,
403,
6933,
8973,
198,
220,
220,
220,
220,
220,
220,
220,
329,
42584,
287,
14631,
9078,
20255,
1600,
366,
403,
6933,
1600,
366,
259,
13658,
8973,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
20218,
796,
15690,
90,
7149,
92,
7,
917,
891,
11,
357,
1120,
11,
642,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4693,
62,
4868,
796,
3127,
62,
19334,
7,
1120,
11,
46792,
38381,
16,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4693,
62,
4868,
796,
41216,
62,
8482,
296,
562,
7,
35448,
62,
4868,
11,
42584,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
329,
479,
287,
352,
25,
7857,
7,
35448,
62,
4868,
11,
352,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
20218,
58,
74,
11,
352,
60,
796,
46792,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
20218,
58,
74,
11,
362,
60,
796,
42584,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
20218,
58,
74,
11,
513,
60,
796,
4693,
62,
4868,
58,
74,
11,
1767,
62,
7857,
62,
1930,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
20218,
58,
74,
11,
604,
60,
796,
4693,
62,
4868,
58,
74,
11,
20018,
62,
13602,
4122,
62,
1930,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
20218,
58,
74,
11,
642,
60,
796,
4693,
62,
4868,
58,
74,
11,
26433,
62,
4906,
62,
1930,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
46792,
62,
8482,
296,
562,
62,
5589,
58,
7,
73,
25,
73,
10,
2920,
828,
1058,
60,
796,
20218,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
474,
796,
474,
1343,
2026,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
220,
198,
437,
220,
198,
198,
2,
1210,
7177,
656,
1366,
14535,
220,
198,
7527,
1108,
62,
8482,
296,
562,
62,
5589,
796,
6060,
19778,
7,
198,
220,
220,
220,
46792,
62,
8482,
296,
562,
62,
5589,
11,
220,
198,
220,
220,
220,
685,
25,
7527,
1108,
62,
43358,
11,
1058,
8482,
296,
562,
62,
43358,
11,
1058,
2618,
62,
7857,
11,
1058,
8482,
296,
562,
11,
1058,
2398,
62,
4906,
60,
198,
8,
198,
198,
2,
787,
21528,
286,
262,
860,
33510,
46111,
4770,
855,
198,
198,
2,
1353,
12,
23701,
1222,
27944,
198,
4852,
23701,
62,
79,
563,
321,
312,
62,
7890,
796,
6060,
19778,
7,
24455,
7,
198,
220,
220,
220,
357,
808,
4613,
5752,
13,
7527,
1108,
62,
43358,
6624,
366,
4852,
12,
23701,
1,
11405,
220,
198,
220,
220,
220,
5752,
13,
8482,
296,
562,
62,
43358,
6624,
366,
9078,
20255,
12340,
220,
198,
220,
220,
220,
46792,
62,
8482,
296,
562,
62,
5589,
198,
4008,
198,
4852,
23701,
62,
79,
563,
321,
312,
62,
29487,
796,
2488,
7568,
1353,
23701,
62,
79,
563,
321,
312,
62,
7890,
7110,
7,
198,
220,
220,
220,
1058,
2618,
62,
7857,
11,
220,
198,
220,
220,
220,
1058,
8482,
296,
562,
11,
220,
198,
220,
220,
220,
1448,
796,
1058,
2398,
62,
4906,
11,
198,
220,
220,
220,
1055,
6386,
2981,
796,
1058,
1416,
1436,
11,
198,
220,
220,
220,
2124,
18242,
796,
366,
2618,
2546,
1600,
198,
220,
220,
220,
331,
18242,
796,
366,
8482,
296,
562,
1600,
198,
220,
220,
220,
8177,
796,
3991,
198,
220,
220,
220,
1303,
7839,
796,
366,
14868,
1108,
796,
1353,
12,
23701,
11,
42584,
796,
27944,
1,
198,
8,
198,
2,
1353,
12,
23701,
1222,
8187,
220,
198,
4852,
23701,
62,
403,
6933,
62,
7890,
796,
8106,
7,
198,
220,
220,
220,
357,
808,
4613,
5752,
13,
7527,
1108,
62,
43358,
6624,
366,
4852,
12,
23701,
1,
11405,
220,
198,
220,
220,
220,
5752,
13,
8482,
296,
562,
62,
43358,
6624,
366,
403,
6933,
12340,
220,
198,
220,
220,
220,
46792,
62,
8482,
296,
562,
62,
5589,
198,
8,
198,
4852,
23701,
62,
403,
6933,
62,
29487,
796,
2488,
7568,
1353,
23701,
62,
403,
6933,
62,
7890,
7110,
7,
198,
220,
220,
220,
1058,
2618,
62,
7857,
11,
220,
198,
220,
220,
220,
1058,
8482,
296,
562,
11,
220,
198,
220,
220,
220,
1448,
796,
1058,
2398,
62,
4906,
11,
198,
220,
220,
220,
1055,
6386,
2981,
796,
1058,
1416,
1436,
11,
198,
220,
220,
220,
2124,
18242,
796,
366,
2618,
2546,
1600,
198,
220,
220,
220,
331,
18242,
796,
366,
8482,
296,
562,
1600,
198,
220,
220,
220,
8177,
796,
3991,
198,
220,
220,
220,
1303,
7839,
796,
366,
14868,
1108,
796,
1353,
12,
23701,
11,
42584,
796,
8187,
1,
198,
8,
198,
2,
1353,
12,
23701,
290,
37204,
220,
198,
4852,
23701,
62,
259,
13658,
62,
7890,
796,
8106,
7,
198,
220,
220,
220,
357,
808,
4613,
5752,
13,
7527,
1108,
62,
43358,
6624,
366,
4852,
12,
23701,
1,
11405,
220,
198,
220,
220,
220,
5752,
13,
8482,
296,
562,
62,
43358,
6624,
366,
259,
13658,
12340,
220,
198,
220,
220,
220,
46792,
62,
8482,
296,
562,
62,
5589,
198,
8,
198,
4852,
23701,
62,
259,
13658,
62,
29487,
796,
2488,
7568,
1353,
23701,
62,
259,
13658,
62,
7890,
7110,
7,
198,
220,
220,
220,
1058,
2618,
62,
7857,
11,
220,
198,
220,
220,
220,
1058,
8482,
296,
562,
11,
220,
198,
220,
220,
220,
1448,
796,
1058,
2398,
62,
4906,
11,
198,
220,
220,
220,
1055,
6386,
2981,
796,
1058,
1416,
1436,
11,
198,
220,
220,
220,
2124,
18242,
796,
366,
2618,
2546,
1600,
198,
220,
220,
220,
331,
18242,
796,
366,
8482,
296,
562,
1600,
198,
220,
220,
220,
8177,
796,
3991,
198,
220,
220,
220,
1303,
7839,
796,
366,
14868,
1108,
796,
1353,
12,
23701,
11,
42584,
796,
37204,
1,
198,
8,
198,
2,
8187,
290,
27944,
220,
198,
403,
6933,
62,
9078,
20255,
62,
7890,
796,
8106,
7,
198,
220,
220,
220,
357,
808,
4613,
5752,
13,
7527,
1108,
62,
43358,
6624,
366,
403,
6933,
1,
11405,
220,
198,
220,
220,
220,
5752,
13,
8482,
296,
562,
62,
43358,
6624,
366,
9078,
20255,
12340,
220,
198,
220,
220,
220,
46792,
62,
8482,
296,
562,
62,
5589,
198,
8,
198,
403,
6933,
62,
9078,
20255,
62,
29487,
796,
2488,
7568,
8187,
62,
9078,
20255,
62,
7890,
7110,
7,
198,
220,
220,
220,
1058,
2618,
62,
7857,
11,
220,
198,
220,
220,
220,
1058,
8482,
296,
562,
11,
220,
198,
220,
220,
220,
1448,
796,
1058,
2398,
62,
4906,
11,
198,
220,
220,
220,
1055,
6386,
2981,
796,
1058,
1416,
1436,
11,
198,
220,
220,
220,
2124,
18242,
796,
366,
2618,
2546,
1600,
198,
220,
220,
220,
331,
18242,
796,
366,
8482,
296,
562,
1600,
198,
220,
220,
220,
8177,
796,
3991,
198,
220,
220,
220,
1303,
7839,
796,
366,
14868,
1108,
796,
8187,
11,
42584,
796,
27944,
1,
198,
8,
198,
2,
8187,
290,
8187,
220,
198,
403,
6933,
62,
403,
6933,
62,
7890,
796,
8106,
7,
198,
220,
220,
220,
357,
808,
4613,
5752,
13,
7527,
1108,
62,
43358,
6624,
366,
403,
6933,
1,
11405,
220,
198,
220,
220,
220,
5752,
13,
8482,
296,
562,
62,
43358,
6624,
366,
403,
6933,
12340,
220,
198,
220,
220,
220,
46792,
62,
8482,
296,
562,
62,
5589,
198,
8,
198,
403,
6933,
62,
403,
6933,
62,
29487,
796,
2488,
7568,
8187,
62,
403,
6933,
62,
7890,
7110,
7,
198,
220,
220,
220,
1058,
2618,
62,
7857,
11,
220,
198,
220,
220,
220,
1058,
8482,
296,
562,
11,
220,
198,
220,
220,
220,
1448,
796,
1058,
2398,
62,
4906,
11,
198,
220,
220,
220,
1055,
6386,
2981,
796,
1058,
1416,
1436,
11,
198,
220,
220,
220,
2124,
18242,
796,
366,
2618,
2546,
1600,
198,
220,
220,
220,
331,
18242,
796,
366,
8482,
296,
562,
1600,
198,
220,
220,
220,
8177,
796,
3991,
198,
220,
220,
220,
1303,
7839,
796,
366,
14868,
1108,
796,
8187,
11,
42584,
796,
8187,
1,
198,
8,
198,
2,
8187,
290,
37204,
220,
198,
403,
6933,
62,
259,
13658,
62,
7890,
796,
8106,
7,
198,
220,
220,
220,
357,
808,
4613,
5752,
13,
7527,
1108,
62,
43358,
6624,
366,
403,
6933,
1,
11405,
220,
198,
220,
220,
220,
5752,
13,
8482,
296,
562,
62,
43358,
6624,
366,
259,
13658,
12340,
220,
198,
220,
220,
220,
46792,
62,
8482,
296,
562,
62,
5589,
198,
8,
198,
403,
6933,
62,
259,
13658,
62,
29487,
796,
2488,
7568,
8187,
62,
259,
13658,
62,
7890,
7110,
7,
198,
220,
220,
220,
1058,
2618,
62,
7857,
11,
220,
198,
220,
220,
220,
1058,
8482,
296,
562,
11,
220,
198,
220,
220,
220,
1448,
796,
1058,
2398,
62,
4906,
11,
198,
220,
220,
220,
1055,
6386,
2981,
796,
1058,
1416,
1436,
11,
198,
220,
220,
220,
2124,
18242,
796,
366,
2618,
2546,
1600,
198,
220,
220,
220,
331,
18242,
796,
366,
8482,
296,
562,
1600,
198,
220,
220,
220,
8177,
796,
3991,
198,
220,
220,
220,
1303,
7839,
796,
366,
14868,
1108,
796,
8187,
11,
42584,
796,
37204,
1,
198,
8,
198,
2,
4220,
12,
23701,
290,
27944,
220,
198,
22487,
23701,
62,
79,
563,
321,
312,
62,
7890,
796,
8106,
7,
198,
220,
220,
220,
357,
808,
4613,
5752,
13,
7527,
1108,
62,
43358,
6624,
366,
22487,
12,
23701,
1,
11405,
220,
198,
220,
220,
220,
5752,
13,
8482,
296,
562,
62,
43358,
6624,
366,
9078,
20255,
12340,
220,
198,
220,
220,
220,
46792,
62,
8482,
296,
562,
62,
5589,
198,
8,
198,
22487,
23701,
62,
79,
563,
321,
312,
62,
29487,
796,
2488,
7568,
4220,
23701,
62,
79,
563,
321,
312,
62,
7890,
7110,
7,
198,
220,
220,
220,
1058,
2618,
62,
7857,
11,
220,
198,
220,
220,
220,
1058,
8482,
296,
562,
11,
220,
198,
220,
220,
220,
1448,
796,
1058,
2398,
62,
4906,
11,
198,
220,
220,
220,
1055,
6386,
2981,
796,
1058,
1416,
1436,
11,
198,
220,
220,
220,
2124,
18242,
796,
366,
2618,
2546,
1600,
198,
220,
220,
220,
331,
18242,
796,
366,
8482,
296,
562,
1600,
198,
220,
220,
220,
8177,
796,
3991,
198,
220,
220,
220,
1303,
7839,
796,
366,
14868,
1108,
796,
4220,
12,
23701,
11,
42584,
796,
27944,
1,
198,
8,
198,
2,
4220,
12,
23701,
290,
8187,
220,
198,
22487,
23701,
62,
403,
6933,
62,
7890,
796,
8106,
7,
198,
220,
220,
220,
357,
808,
4613,
5752,
13,
7527,
1108,
62,
43358,
6624,
366,
22487,
12,
23701,
1,
11405,
220,
198,
220,
220,
220,
5752,
13,
8482,
296,
562,
62,
43358,
6624,
366,
403,
6933,
12340,
220,
198,
220,
220,
220,
46792,
62,
8482,
296,
562,
62,
5589,
198,
8,
198,
22487,
23701,
62,
403,
6933,
62,
29487,
796,
2488,
7568,
4220,
23701,
62,
403,
6933,
62,
7890,
7110,
7,
198,
220,
220,
220,
1058,
2618,
62,
7857,
11,
220,
198,
220,
220,
220,
1058,
8482,
296,
562,
11,
220,
198,
220,
220,
220,
1448,
796,
1058,
2398,
62,
4906,
11,
198,
220,
220,
220,
1055,
6386,
2981,
796,
1058,
1416,
1436,
11,
198,
220,
220,
220,
2124,
18242,
796,
366,
2618,
2546,
1600,
198,
220,
220,
220,
331,
18242,
796,
366,
8482,
296,
562,
1600,
198,
220,
220,
220,
8177,
796,
3991,
198,
220,
220,
220,
1303,
7839,
796,
366,
14868,
1108,
796,
4220,
12,
23701,
11,
42584,
796,
8187,
1,
198,
8,
198,
2,
4220,
12,
23701,
290,
37204,
220,
198,
22487,
23701,
62,
259,
13658,
62,
7890,
796,
8106,
7,
198,
220,
220,
220,
357,
808,
4613,
5752,
13,
7527,
1108,
62,
43358,
6624,
366,
22487,
12,
23701,
1,
11405,
220,
198,
220,
220,
220,
5752,
13,
8482,
296,
562,
62,
43358,
6624,
366,
259,
13658,
12340,
220,
198,
220,
220,
220,
46792,
62,
8482,
296,
562,
62,
5589,
198,
8,
198,
22487,
23701,
62,
259,
13658,
62,
29487,
796,
2488,
7568,
4220,
23701,
62,
259,
13658,
62,
7890,
7110,
7,
198,
220,
220,
220,
1058,
2618,
62,
7857,
11,
220,
198,
220,
220,
220,
1058,
8482,
296,
562,
11,
220,
198,
220,
220,
220,
1448,
796,
1058,
2398,
62,
4906,
11,
198,
220,
220,
220,
1055,
6386,
2981,
796,
1058,
1416,
1436,
11,
198,
220,
220,
220,
2124,
18242,
796,
366,
2618,
2546,
1600,
198,
220,
220,
220,
331,
18242,
796,
366,
8482,
296,
562,
1600,
198,
220,
220,
220,
1303,
7839,
796,
366,
14868,
1108,
796,
4220,
12,
23701,
11,
42584,
796,
37204,
1600,
198,
220,
220,
220,
8177,
796,
3991,
198,
8,
198,
2,
1234,
477,
21528,
1978,
198,
439,
62,
1477,
7916,
62,
489,
1747,
796,
1345,
1747,
13,
29487,
7,
198,
220,
220,
220,
1353,
23701,
62,
79,
563,
321,
312,
62,
29487,
11,
220,
198,
220,
220,
220,
1353,
23701,
62,
403,
6933,
62,
29487,
11,
220,
198,
220,
220,
220,
1353,
23701,
62,
259,
13658,
62,
29487,
11,
198,
220,
220,
220,
8187,
62,
9078,
20255,
62,
29487,
11,
198,
220,
220,
220,
8187,
62,
403,
6933,
62,
29487,
11,
198,
220,
220,
220,
8187,
62,
259,
13658,
62,
29487,
11,
198,
220,
220,
220,
4220,
23701,
62,
79,
563,
321,
312,
62,
29487,
11,
220,
198,
220,
220,
220,
4220,
23701,
62,
403,
6933,
62,
29487,
11,
198,
220,
220,
220,
4220,
23701,
62,
259,
13658,
62,
29487,
11,
198,
220,
220,
220,
12461,
796,
357,
18,
11,
18,
828,
198,
220,
220,
220,
1303,
1455,
437,
796,
3991,
11,
198,
220,
220,
220,
1303,
39786,
796,
1345,
1747,
13,
25928,
7,
18,
11,
18,
11,
23245,
796,
685,
15,
13,
18,
11,
657,
13,
18,
11,
657,
13,
18,
46570,
198,
220,
220,
220,
1303,
87,
18242,
796,
366,
2618,
2546,
1600,
198,
220,
220,
220,
1303,
2645,
9608,
796,
366,
8482,
296,
562,
1,
198,
220,
220,
220,
3670,
796,
14631,
16,
1600,
366,
17,
1600,
366,
18,
1600,
366,
19,
1600,
366,
20,
1600,
366,
21,
1600,
366,
22,
1600,
366,
23,
1600,
366,
24,
8973,
198,
8,
198,
3646,
1747,
13,
21928,
5647,
7,
439,
62,
1477,
7916,
62,
489,
1747,
11,
366,
19571,
5647,
82,
14,
439,
62,
1477,
7916,
62,
489,
1747,
13,
11134,
4943,
198
] | 2.413984 | 2,703 |
########################################
## File Name: control_types.jl
## Author: Haruki Nishimura (hnishimura@stanford.edu)
## Date Created: 2020/05/12
## Description: Control Type Definitions for SACBP
########################################
import Base.vec
abstract type Control end
struct PosControl{T<:Real} <: Control
t::Float64
vel::Vector{T}
dim::Int64
function PosControl{T}(t,vel,dim) where {T<:Real}
if dim != length(vel)
error(ArgumentError("Invalid control vector length."));
else
return new(t,vel,dim);
end
end
end
PosControl(t::Real,vel::Vector{T},dim::Int64=length(vel)) where {T<:Real} = PosControl{T}(t,vel,dim);
PosControl(t::Real,vel::T) where {T<:Real} = PosControl{T}(t,[vel],1);
vec(u::PosControl) = u.vel;
struct MControl2D{T<:Real} <: Control
t::Float64
fx::T # Force x
fy::T # Force y
tr::T # Torque
end
function MControl2D(t::Real,params::Vector{T}) where {T<:Real}
if length(params) != 3
error(ArgumentError("Invalid parameter vector length."))
else
return MControl2D{T}(t,params[1],params[2],params[3]);
end
end
vec(u::MControl2D) = [u.fx,u.fy,u.tr];
| [
29113,
7804,
198,
2235,
9220,
6530,
25,
1630,
62,
19199,
13,
20362,
198,
2235,
6434,
25,
2113,
11308,
48438,
43817,
357,
21116,
680,
43817,
31,
14192,
3841,
13,
15532,
8,
198,
2235,
7536,
15622,
25,
12131,
14,
2713,
14,
1065,
198,
2235,
12489,
25,
6779,
5994,
45205,
329,
311,
2246,
20866,
198,
29113,
7804,
198,
198,
11748,
7308,
13,
35138,
198,
198,
397,
8709,
2099,
6779,
886,
198,
198,
7249,
18574,
15988,
90,
51,
27,
25,
15633,
92,
1279,
25,
6779,
198,
220,
220,
220,
256,
3712,
43879,
2414,
198,
220,
220,
220,
11555,
3712,
38469,
90,
51,
92,
198,
220,
220,
220,
5391,
3712,
5317,
2414,
198,
220,
220,
220,
2163,
18574,
15988,
90,
51,
92,
7,
83,
11,
626,
11,
27740,
8,
810,
1391,
51,
27,
25,
15633,
92,
198,
220,
220,
220,
220,
220,
220,
220,
611,
5391,
14512,
4129,
7,
626,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4049,
7,
28100,
1713,
12331,
7203,
44651,
1630,
15879,
4129,
526,
18125,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1441,
649,
7,
83,
11,
626,
11,
27740,
1776,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
437,
198,
21604,
15988,
7,
83,
3712,
15633,
11,
626,
3712,
38469,
90,
51,
5512,
27740,
3712,
5317,
2414,
28,
13664,
7,
626,
4008,
810,
1391,
51,
27,
25,
15633,
92,
796,
18574,
15988,
90,
51,
92,
7,
83,
11,
626,
11,
27740,
1776,
198,
21604,
15988,
7,
83,
3712,
15633,
11,
626,
3712,
51,
8,
810,
1391,
51,
27,
25,
15633,
92,
796,
18574,
15988,
90,
51,
92,
7,
83,
17414,
626,
4357,
16,
1776,
198,
35138,
7,
84,
3712,
21604,
15988,
8,
796,
334,
13,
626,
26,
628,
198,
7249,
337,
15988,
17,
35,
90,
51,
27,
25,
15633,
92,
1279,
25,
6779,
198,
220,
220,
220,
256,
3712,
43879,
2414,
198,
220,
220,
220,
277,
87,
3712,
51,
220,
1303,
5221,
2124,
198,
220,
220,
220,
277,
88,
3712,
51,
220,
1303,
5221,
331,
198,
220,
220,
220,
491,
3712,
51,
220,
1303,
4022,
4188,
198,
437,
198,
8818,
337,
15988,
17,
35,
7,
83,
3712,
15633,
11,
37266,
3712,
38469,
90,
51,
30072,
810,
1391,
51,
27,
25,
15633,
92,
198,
220,
220,
220,
611,
4129,
7,
37266,
8,
14512,
513,
198,
220,
220,
220,
220,
220,
220,
220,
4049,
7,
28100,
1713,
12331,
7203,
44651,
11507,
15879,
4129,
526,
4008,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
337,
15988,
17,
35,
90,
51,
92,
7,
83,
11,
37266,
58,
16,
4357,
37266,
58,
17,
4357,
37266,
58,
18,
36563,
198,
220,
220,
220,
886,
198,
437,
198,
35138,
7,
84,
3712,
44,
15988,
17,
35,
8,
796,
685,
84,
13,
21373,
11,
84,
13,
24928,
11,
84,
13,
2213,
11208,
198
] | 2.463115 | 488 |
using GenomePermutations
using GenomicFeatures
using Documenter
push!(LOAD_PATH,"../src/")
DocMeta.setdocmeta!(GenomePermutations, :DocTestSetup, :(using GenomePermutations); recursive=true)
makedocs(;
modules=[GenomePermutations],
authors="Simone De Angelis <simone.deangelis@qmul.ac.uk> <side97.11+jl@gmail.com>",
repo="https://github.com/sdangelis/GenomePermutations.jl/blob/{commit}{path}#{line}",
sitename="GenomePermutations.jl",
format=Documenter.HTML(;
prettyurls=get(ENV, "CI", "false") == "true",
canonical="https://sdangelis.github.io/GenomePermutations.jl",
assets=String[],
),
pages=[
"Home" => "index.md",
],
)
deploydocs(;
repo="github.com/sdangelis/GenomePermutations.jl",
devbranch="main",
)
| [
3500,
5215,
462,
5990,
21973,
602,
198,
3500,
5215,
10179,
23595,
198,
3500,
16854,
263,
198,
198,
14689,
0,
7,
35613,
62,
34219,
553,
40720,
10677,
14,
4943,
198,
23579,
48526,
13,
2617,
15390,
28961,
0,
7,
13746,
462,
5990,
21973,
602,
11,
1058,
23579,
14402,
40786,
11,
36147,
3500,
5215,
462,
5990,
21973,
602,
1776,
45115,
28,
7942,
8,
198,
198,
76,
4335,
420,
82,
7,
26,
198,
220,
220,
220,
13103,
41888,
13746,
462,
5990,
21973,
602,
4357,
198,
220,
220,
220,
7035,
2625,
8890,
505,
1024,
3905,
271,
1279,
14323,
505,
13,
2934,
8368,
271,
31,
80,
76,
377,
13,
330,
13,
2724,
29,
1279,
1589,
5607,
13,
1157,
10,
20362,
31,
14816,
13,
785,
29,
1600,
198,
220,
220,
220,
29924,
2625,
5450,
1378,
12567,
13,
785,
14,
21282,
8368,
271,
14,
13746,
462,
5990,
21973,
602,
13,
20362,
14,
2436,
672,
14,
90,
41509,
18477,
6978,
92,
2,
90,
1370,
92,
1600,
198,
220,
220,
220,
1650,
12453,
2625,
13746,
462,
5990,
21973,
602,
13,
20362,
1600,
198,
220,
220,
220,
5794,
28,
24941,
263,
13,
28656,
7,
26,
198,
220,
220,
220,
220,
220,
220,
220,
2495,
6371,
82,
28,
1136,
7,
1677,
53,
11,
366,
25690,
1600,
366,
9562,
4943,
6624,
366,
7942,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
40091,
2625,
5450,
1378,
21282,
8368,
271,
13,
12567,
13,
952,
14,
13746,
462,
5990,
21973,
602,
13,
20362,
1600,
198,
220,
220,
220,
220,
220,
220,
220,
6798,
28,
10100,
58,
4357,
198,
220,
220,
220,
10612,
198,
220,
220,
220,
5468,
41888,
198,
220,
220,
220,
220,
220,
220,
220,
366,
16060,
1,
5218,
366,
9630,
13,
9132,
1600,
198,
220,
220,
220,
16589,
198,
8,
198,
198,
2934,
1420,
31628,
7,
26,
198,
220,
220,
220,
29924,
2625,
12567,
13,
785,
14,
21282,
8368,
271,
14,
13746,
462,
5990,
21973,
602,
13,
20362,
1600,
198,
220,
220,
220,
1614,
1671,
3702,
2625,
12417,
1600,
198,
8,
198
] | 2.382979 | 329 |
# ---
# title: 688. Knight Probability in Chessboard
# id: problem688
# author: Indigo
# date: 2021-06-29
# difficulty: Medium
# categories: Dynamic Programming
# link: <https://leetcode.com/problems/knight-probability-in-chessboard/description/>
# hidden: true
# ---
#
# On an `N`x`N` chessboard, a knight starts at the `r`-th row and `c`-th column
# and attempts to make exactly `K` moves. The rows and columns are 0 indexed, so
# the top-left square is `(0, 0)`, and the bottom-right square is `(N-1, N-1)`.
#
# A chess knight has 8 possible moves it can make, as illustrated below. Each
# move is two squares in a cardinal direction, then one square in an orthogonal
# direction.
#
#
#
# 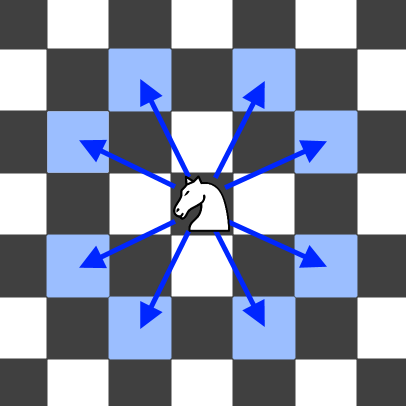
#
#
#
# Each time the knight is to move, it chooses one of eight possible moves
# uniformly at random (even if the piece would go off the chessboard) and moves
# there.
#
# The knight continues moving until it has made exactly `K` moves or has moved
# off the chessboard. Return the probability that the knight remains on the
# board after it has stopped moving.
#
#
#
# **Example:**
#
#
#
# Input: 3, 2, 0, 0
# Output: 0.0625
# Explanation: There are two moves (to (1,2), (2,1)) that will keep the knight on the board.
# From each of those positions, there are also two moves that will keep the knight on the board.
# The total probability the knight stays on the board is 0.0625.
#
#
#
#
# **Note:**
#
# * `N` will be between 1 and 25.
# * `K` will be between 0 and 100.
# * The knight always initially starts on the board.
#
#
## @lc code=start
using LeetCode
function knight_probability(n::Int, k::Int, row::Int, col::Int)
dp = fill(0, n, n, 2)
dp[row + 1, col + 1, 1] = 1
for i in 1:k
dp1 = @view(dp[:, :, mod1(i, 2)])
dp2 = @view(dp[:, :, mod1(i + 1, 2)])
idcs = CartesianIndices(dp2)
for I in idcs, hop in ((1, 2), (2, 1), (-1, 2), (2, -1), (1, -2), (-2, 1), (-1, -2), (-2, -1))
new_I = I + CartesianIndex(hop)
new_I ∈ idcs && (dp2[new_I] += dp1[I])
end
fill!(dp1, 0)
end
return sum(dp) / 8 ^ k
end
## @lc code=end
| [
2,
11420,
198,
2,
3670,
25,
718,
3459,
13,
6700,
30873,
1799,
287,
25774,
3526,
198,
2,
4686,
25,
1917,
34427,
198,
2,
1772,
25,
40673,
198,
2,
3128,
25,
33448,
12,
3312,
12,
1959,
198,
2,
8722,
25,
13398,
198,
2,
9376,
25,
26977,
30297,
198,
2,
2792,
25,
1279,
5450,
1378,
293,
316,
8189,
13,
785,
14,
1676,
22143,
14,
74,
3847,
12,
1676,
65,
1799,
12,
259,
12,
2395,
824,
3526,
14,
11213,
15913,
198,
2,
7104,
25,
2081,
198,
2,
11420,
198,
2,
220,
198,
2,
1550,
281,
4600,
45,
63,
87,
63,
45,
63,
19780,
3526,
11,
257,
22062,
4940,
379,
262,
4600,
81,
63,
12,
400,
5752,
290,
4600,
66,
63,
12,
400,
5721,
198,
2,
290,
6370,
284,
787,
3446,
4600,
42,
63,
6100,
13,
383,
15274,
290,
15180,
389,
657,
41497,
11,
523,
198,
2,
262,
1353,
12,
9464,
6616,
318,
4600,
7,
15,
11,
657,
8,
47671,
290,
262,
4220,
12,
3506,
6616,
318,
4600,
7,
45,
12,
16,
11,
399,
12,
16,
8,
44646,
198,
2,
220,
198,
2,
317,
19780,
22062,
468,
807,
1744,
6100,
340,
460,
787,
11,
355,
18542,
2174,
13,
5501,
198,
2,
1445,
318,
734,
24438,
287,
257,
38691,
4571,
11,
788,
530,
6616,
287,
281,
29617,
519,
20996,
198,
2,
4571,
13,
198,
2,
220,
198,
2,
220,
198,
2,
220,
198,
2,
5145,
58,
16151,
5450,
1378,
19668,
13,
293,
316,
8189,
13,
785,
14,
39920,
14,
7908,
14,
940,
14,
1065,
14,
74,
3847,
13,
11134,
8,
198,
2,
220,
198,
2,
220,
198,
2,
220,
198,
2,
5501,
640,
262,
22062,
318,
284,
1445,
11,
340,
19769,
530,
286,
3624,
1744,
6100,
198,
2,
42096,
379,
4738,
357,
10197,
611,
262,
3704,
561,
467,
572,
262,
19780,
3526,
8,
290,
6100,
198,
2,
612,
13,
198,
2,
220,
198,
2,
383,
22062,
4477,
3867,
1566,
340,
468,
925,
3446,
4600,
42,
63,
6100,
393,
468,
3888,
198,
2,
572,
262,
19780,
3526,
13,
8229,
262,
12867,
326,
262,
22062,
3793,
319,
262,
198,
2,
3096,
706,
340,
468,
5025,
3867,
13,
198,
2,
220,
198,
2,
220,
198,
2,
220,
198,
2,
12429,
16281,
25,
1174,
198,
2,
220,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
220,
220,
220,
23412,
25,
513,
11,
362,
11,
657,
11,
657,
198,
2,
220,
220,
220,
220,
25235,
25,
657,
13,
3312,
1495,
198,
2,
220,
220,
220,
220,
50125,
341,
25,
1318,
389,
734,
6100,
357,
1462,
357,
16,
11,
17,
828,
357,
17,
11,
16,
4008,
326,
481,
1394,
262,
22062,
319,
262,
3096,
13,
198,
2,
220,
220,
220,
220,
3574,
1123,
286,
883,
6116,
11,
612,
389,
635,
734,
6100,
326,
481,
1394,
262,
22062,
319,
262,
3096,
13,
198,
2,
220,
220,
220,
220,
383,
2472,
12867,
262,
22062,
14768,
319,
262,
3096,
318,
657,
13,
3312,
1495,
13,
198,
2,
220,
220,
220,
220,
220,
198,
2,
220,
198,
2,
220,
198,
2,
220,
198,
2,
12429,
6425,
25,
1174,
198,
2,
220,
198,
2,
220,
220,
1635,
4600,
45,
63,
481,
307,
1022,
352,
290,
1679,
13,
198,
2,
220,
220,
1635,
4600,
42,
63,
481,
307,
1022,
657,
290,
1802,
13,
198,
2,
220,
220,
1635,
383,
22062,
1464,
7317,
4940,
319,
262,
3096,
13,
198,
2,
220,
198,
2,
220,
198,
2235,
2488,
44601,
2438,
28,
9688,
198,
3500,
1004,
316,
10669,
198,
198,
8818,
22062,
62,
1676,
65,
1799,
7,
77,
3712,
5317,
11,
479,
3712,
5317,
11,
5752,
3712,
5317,
11,
951,
3712,
5317,
8,
198,
220,
220,
220,
288,
79,
796,
6070,
7,
15,
11,
299,
11,
299,
11,
362,
8,
198,
220,
220,
220,
288,
79,
58,
808,
1343,
352,
11,
951,
1343,
352,
11,
352,
60,
796,
352,
198,
220,
220,
220,
329,
1312,
287,
352,
25,
74,
198,
220,
220,
220,
220,
220,
220,
220,
288,
79,
16,
796,
2488,
1177,
7,
26059,
58,
45299,
1058,
11,
953,
16,
7,
72,
11,
362,
8,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
288,
79,
17,
796,
2488,
1177,
7,
26059,
58,
45299,
1058,
11,
953,
16,
7,
72,
1343,
352,
11,
362,
8,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
4686,
6359,
796,
13690,
35610,
5497,
1063,
7,
26059,
17,
8,
198,
220,
220,
220,
220,
220,
220,
220,
329,
314,
287,
4686,
6359,
11,
1725,
287,
14808,
16,
11,
362,
828,
357,
17,
11,
352,
828,
13841,
16,
11,
362,
828,
357,
17,
11,
532,
16,
828,
357,
16,
11,
532,
17,
828,
13841,
17,
11,
352,
828,
13841,
16,
11,
532,
17,
828,
13841,
17,
11,
532,
16,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
62,
40,
796,
314,
1343,
13690,
35610,
15732,
7,
8548,
8,
220,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
649,
62,
40,
18872,
230,
4686,
6359,
11405,
357,
26059,
17,
58,
3605,
62,
40,
60,
15853,
288,
79,
16,
58,
40,
12962,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
6070,
0,
7,
26059,
16,
11,
657,
8,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
2160,
7,
26059,
8,
1220,
807,
10563,
479,
198,
437,
198,
2235,
2488,
44601,
2438,
28,
437,
198
] | 2.464835 | 910 |
using Test
using NCDatasets
using DataStructures
function example_file(i,array)
fname = tempname()
@debug "fname $fname"
Dataset(fname,"c") do ds
# Dimensions
ds.dim["lon"] = size(array,1)
ds.dim["lat"] = size(array,2)
ds.dim["time"] = Inf
# Declare variables
ncvar = defVar(ds,"var", Float64, ("lon", "lat", "time"))
ncvar.attrib["field"] = "u-wind, scalar, series"
ncvar.attrib["units"] = "meter second-1"
ncvar.attrib["long_name"] = "surface u-wind component"
ncvar.attrib["time"] = "time"
ncvar.attrib["coordinates"] = "lon lat"
nclat = defVar(ds,"lat", Float64, ("lat",))
nclat.attrib["units"] = "degrees_north"
nclon = defVar(ds,"lon", Float64, ("lon",))
nclon.attrib["units"] = "degrees_east"
nclon.attrib["modulo"] = 360.0
nctime = defVar(ds,"time", Float64, ("time",))
nctime.attrib["long_name"] = "surface wind time"
nctime.attrib["field"] = "time, scalar, series"
nctime.attrib["units"] = "days since 2000-01-01 00:00:00 GMT"
# Global attributes
ds.attrib["history"] = "foo"
# Define variables
g = defGroup(ds,"mygroup")
ncvarg = defVar(g,"var", Float64, ("lon", "lat", "time"))
ncvarg.attrib["field"] = "u-wind, scalar, series"
ncvarg.attrib["units"] = "meter second-1"
ncvarg.attrib["long_name"] = "surface u-wind component"
ncvarg.attrib["time"] = "time"
ncvarg.attrib["coordinates"] = "lon lat"
ncvar[:,:,1] = array
ncvarg[:,:,1] = array.+1
#nclon[:] = 1:size(array,1)
#nclat[:] = 1:size(array,2)
nctime[:] = i
end
return fname
end
A = randn(2,3,1)
fname = example_file(1,A)
ds = Dataset(fname)
info = NCDatasets.metadata(ds)
#@show info
dds = DeferDataset(fname);
varname = "var"
datavar = variable(dds,varname);
data = datavar[:,:,:]
@test A == datavar[:,:,:]
@test dds.attrib["history"] == "foo"
@test datavar.attrib["units"] == "meter second-1"
@test dimnames(datavar) == ("lon", "lat", "time")
lon = variable(dds,"lon");
@test lon.attrib["units"] == "degrees_east"
@test size(lon) == (size(data,1),)
datavar = dds[varname]
@test A == datavar[:,:,:]
@test dimnames(datavar) == ("lon", "lat", "time")
@test dds.dim["lon"] == size(A,1)
@test dds.dim["lat"] == size(A,2)
@test dds.dim["time"] == size(A,3)
@test dds.dim["time"] == size(A,3)
close(dds)
# show
dds_buf = IOBuffer()
show(dds_buf,dds)
ds_buf = IOBuffer()
show(ds_buf,dds)
@test String(take!(dds_buf)) == String(take!(ds_buf))
#=
# write
dds = Dataset(fnames,"a");
dds["datavar"][2,2,:] = 1:length(fnames)
for n = 1:length(fnames)
Dataset(fnames[n]) do ds
@test ds["datavar"][2,2,1] == n
end
end
dds.attrib["history"] = "foo2"
sync(dds)
Dataset(fnames[1]) do ds
@test ds.attrib["history"] == "foo2"
end
@test_throws NCDatasets.NetCDFError Dataset(fnames,"not-a-mode")
@test keys(dds) == ["datavar", "lat", "lon", "time"]
@test keys(dds.dim) == ["lon", "lat", "time"]
@test NCDatasets.groupname(dds) == "/"
@test size(dds["var"]) == (2, 3, 3)
@test size(dds["var"].var) == (2, 3, 3)
@test name(dds["var"].var) == "var"
@test NCDatasets.groupname(dds.group["mygroup"]) == "mygroup"
# create new dimension in all files
dds.dim["newdim"] = 123;
sync(dds);
Dataset(fnames[1]) do ds
@test ds.dim["newdim"] == 123
end
close(dds)
=#
nothing
| [
3500,
6208,
198,
3500,
399,
8610,
265,
292,
1039,
198,
3500,
6060,
44909,
942,
198,
198,
8818,
1672,
62,
7753,
7,
72,
11,
18747,
8,
198,
220,
220,
220,
277,
3672,
796,
20218,
3672,
3419,
198,
220,
220,
220,
2488,
24442,
366,
69,
3672,
720,
69,
3672,
1,
628,
220,
220,
220,
16092,
292,
316,
7,
69,
3672,
553,
66,
4943,
466,
288,
82,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
41265,
628,
220,
220,
220,
220,
220,
220,
220,
288,
82,
13,
27740,
14692,
14995,
8973,
796,
2546,
7,
18747,
11,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
288,
82,
13,
27740,
14692,
15460,
8973,
796,
2546,
7,
18747,
11,
17,
8,
198,
220,
220,
220,
220,
220,
220,
220,
288,
82,
13,
27740,
14692,
2435,
8973,
796,
4806,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
16691,
533,
9633,
628,
220,
220,
220,
220,
220,
220,
220,
299,
66,
7785,
796,
825,
19852,
7,
9310,
553,
7785,
1600,
48436,
2414,
11,
5855,
14995,
1600,
366,
15460,
1600,
366,
2435,
48774,
198,
220,
220,
220,
220,
220,
220,
220,
299,
66,
7785,
13,
1078,
822,
14692,
3245,
8973,
796,
366,
84,
12,
7972,
11,
16578,
283,
11,
2168,
1,
198,
220,
220,
220,
220,
220,
220,
220,
299,
66,
7785,
13,
1078,
822,
14692,
41667,
8973,
796,
366,
27231,
1218,
12,
16,
1,
198,
220,
220,
220,
220,
220,
220,
220,
299,
66,
7785,
13,
1078,
822,
14692,
6511,
62,
3672,
8973,
796,
366,
42029,
334,
12,
7972,
7515,
1,
198,
220,
220,
220,
220,
220,
220,
220,
299,
66,
7785,
13,
1078,
822,
14692,
2435,
8973,
796,
366,
2435,
1,
198,
220,
220,
220,
220,
220,
220,
220,
299,
66,
7785,
13,
1078,
822,
14692,
37652,
17540,
8973,
796,
366,
14995,
3042,
1,
628,
198,
220,
220,
220,
220,
220,
220,
220,
299,
565,
265,
796,
825,
19852,
7,
9310,
553,
15460,
1600,
48436,
2414,
11,
5855,
15460,
1600,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
299,
565,
265,
13,
1078,
822,
14692,
41667,
8973,
796,
366,
13500,
6037,
62,
43588,
1,
628,
220,
220,
220,
220,
220,
220,
220,
299,
565,
261,
796,
825,
19852,
7,
9310,
553,
14995,
1600,
48436,
2414,
11,
5855,
14995,
1600,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
299,
565,
261,
13,
1078,
822,
14692,
41667,
8973,
796,
366,
13500,
6037,
62,
23316,
1,
198,
220,
220,
220,
220,
220,
220,
220,
299,
565,
261,
13,
1078,
822,
14692,
4666,
43348,
8973,
796,
11470,
13,
15,
628,
220,
220,
220,
220,
220,
220,
220,
299,
310,
524,
796,
825,
19852,
7,
9310,
553,
2435,
1600,
48436,
2414,
11,
5855,
2435,
1600,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
299,
310,
524,
13,
1078,
822,
14692,
6511,
62,
3672,
8973,
796,
366,
42029,
2344,
640,
1,
198,
220,
220,
220,
220,
220,
220,
220,
299,
310,
524,
13,
1078,
822,
14692,
3245,
8973,
796,
366,
2435,
11,
16578,
283,
11,
2168,
1,
198,
220,
220,
220,
220,
220,
220,
220,
299,
310,
524,
13,
1078,
822,
14692,
41667,
8973,
796,
366,
12545,
1201,
4751,
12,
486,
12,
486,
3571,
25,
405,
25,
405,
16987,
1,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
8060,
12608,
628,
220,
220,
220,
220,
220,
220,
220,
288,
82,
13,
1078,
822,
14692,
23569,
8973,
796,
366,
21943,
1,
628,
220,
220,
220,
220,
220,
220,
220,
1303,
2896,
500,
9633,
628,
220,
220,
220,
220,
220,
220,
220,
308,
796,
825,
13247,
7,
9310,
553,
1820,
8094,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
299,
33967,
853,
796,
825,
19852,
7,
70,
553,
7785,
1600,
48436,
2414,
11,
5855,
14995,
1600,
366,
15460,
1600,
366,
2435,
48774,
198,
220,
220,
220,
220,
220,
220,
220,
299,
33967,
853,
13,
1078,
822,
14692,
3245,
8973,
796,
366,
84,
12,
7972,
11,
16578,
283,
11,
2168,
1,
198,
220,
220,
220,
220,
220,
220,
220,
299,
33967,
853,
13,
1078,
822,
14692,
41667,
8973,
796,
366,
27231,
1218,
12,
16,
1,
198,
220,
220,
220,
220,
220,
220,
220,
299,
33967,
853,
13,
1078,
822,
14692,
6511,
62,
3672,
8973,
796,
366,
42029,
334,
12,
7972,
7515,
1,
198,
220,
220,
220,
220,
220,
220,
220,
299,
33967,
853,
13,
1078,
822,
14692,
2435,
8973,
796,
366,
2435,
1,
198,
220,
220,
220,
220,
220,
220,
220,
299,
33967,
853,
13,
1078,
822,
14692,
37652,
17540,
8973,
796,
366,
14995,
3042,
1,
628,
220,
220,
220,
220,
220,
220,
220,
299,
66,
7785,
58,
45299,
45299,
16,
60,
796,
7177,
198,
220,
220,
220,
220,
220,
220,
220,
299,
33967,
853,
58,
45299,
45299,
16,
60,
796,
7177,
13,
10,
16,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
77,
565,
261,
58,
47715,
796,
352,
25,
7857,
7,
18747,
11,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
77,
565,
265,
58,
47715,
796,
352,
25,
7857,
7,
18747,
11,
17,
8,
198,
220,
220,
220,
220,
220,
220,
220,
299,
310,
524,
58,
47715,
796,
1312,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
277,
3672,
198,
437,
198,
198,
32,
796,
43720,
77,
7,
17,
11,
18,
11,
16,
8,
628,
198,
69,
3672,
796,
1672,
62,
7753,
7,
16,
11,
32,
8,
628,
198,
9310,
796,
16092,
292,
316,
7,
69,
3672,
8,
198,
198,
10951,
796,
399,
8610,
265,
292,
1039,
13,
38993,
7,
9310,
8,
198,
198,
2,
31,
12860,
7508,
198,
198,
33714,
796,
2896,
263,
27354,
292,
316,
7,
69,
3672,
1776,
198,
85,
1501,
480,
796,
366,
7785,
1,
198,
19608,
615,
283,
796,
7885,
7,
33714,
11,
85,
1501,
480,
1776,
198,
7890,
796,
4818,
615,
283,
58,
45299,
45299,
47715,
628,
198,
31,
9288,
317,
6624,
4818,
615,
283,
58,
45299,
45299,
47715,
198,
198,
31,
9288,
288,
9310,
13,
1078,
822,
14692,
23569,
8973,
6624,
366,
21943,
1,
198,
31,
9288,
4818,
615,
283,
13,
1078,
822,
14692,
41667,
8973,
6624,
366,
27231,
1218,
12,
16,
1,
198,
198,
31,
9288,
5391,
14933,
7,
19608,
615,
283,
8,
6624,
5855,
14995,
1600,
366,
15460,
1600,
366,
2435,
4943,
198,
14995,
796,
7885,
7,
33714,
553,
14995,
15341,
198,
31,
9288,
300,
261,
13,
1078,
822,
14692,
41667,
8973,
6624,
366,
13500,
6037,
62,
23316,
1,
198,
31,
9288,
2546,
7,
14995,
8,
6624,
357,
7857,
7,
7890,
11,
16,
828,
8,
628,
198,
19608,
615,
283,
796,
288,
9310,
58,
85,
1501,
480,
60,
198,
31,
9288,
317,
6624,
4818,
615,
283,
58,
45299,
45299,
47715,
198,
198,
31,
9288,
5391,
14933,
7,
19608,
615,
283,
8,
6624,
5855,
14995,
1600,
366,
15460,
1600,
366,
2435,
4943,
198,
198,
31,
9288,
288,
9310,
13,
27740,
14692,
14995,
8973,
6624,
2546,
7,
32,
11,
16,
8,
198,
31,
9288,
288,
9310,
13,
27740,
14692,
15460,
8973,
6624,
2546,
7,
32,
11,
17,
8,
198,
31,
9288,
288,
9310,
13,
27740,
14692,
2435,
8973,
6624,
2546,
7,
32,
11,
18,
8,
198,
198,
31,
9288,
288,
9310,
13,
27740,
14692,
2435,
8973,
6624,
2546,
7,
32,
11,
18,
8,
198,
19836,
7,
33714,
8,
198,
198,
2,
905,
198,
33714,
62,
29325,
796,
314,
9864,
13712,
3419,
198,
12860,
7,
33714,
62,
29325,
11,
33714,
8,
198,
198,
9310,
62,
29325,
796,
314,
9864,
13712,
3419,
198,
12860,
7,
9310,
62,
29325,
11,
33714,
8,
198,
198,
31,
9288,
10903,
7,
20657,
0,
7,
33714,
62,
29325,
4008,
6624,
10903,
7,
20657,
0,
7,
9310,
62,
29325,
4008,
198,
198,
2,
28,
198,
2,
3551,
198,
33714,
796,
16092,
292,
316,
7,
69,
14933,
553,
64,
15341,
198,
33714,
14692,
19608,
615,
283,
1,
7131,
17,
11,
17,
11,
47715,
796,
352,
25,
13664,
7,
69,
14933,
8,
198,
198,
1640,
299,
796,
352,
25,
13664,
7,
69,
14933,
8,
198,
220,
220,
220,
16092,
292,
316,
7,
69,
14933,
58,
77,
12962,
466,
288,
82,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
288,
82,
14692,
19608,
615,
283,
1,
7131,
17,
11,
17,
11,
16,
60,
6624,
299,
198,
220,
220,
220,
886,
198,
437,
198,
198,
33714,
13,
1078,
822,
14692,
23569,
8973,
796,
366,
21943,
17,
1,
198,
27261,
7,
33714,
8,
198,
198,
27354,
292,
316,
7,
69,
14933,
58,
16,
12962,
466,
288,
82,
198,
220,
220,
220,
2488,
9288,
288,
82,
13,
1078,
822,
14692,
23569,
8973,
6624,
366,
21943,
17,
1,
198,
437,
198,
198,
31,
9288,
62,
400,
8516,
399,
8610,
265,
292,
1039,
13,
7934,
34,
8068,
12331,
16092,
292,
316,
7,
69,
14933,
553,
1662,
12,
64,
12,
14171,
4943,
198,
198,
31,
9288,
8251,
7,
33714,
8,
6624,
14631,
19608,
615,
283,
1600,
366,
15460,
1600,
366,
14995,
1600,
366,
2435,
8973,
198,
31,
9288,
8251,
7,
33714,
13,
27740,
8,
6624,
14631,
14995,
1600,
366,
15460,
1600,
366,
2435,
8973,
198,
31,
9288,
399,
8610,
265,
292,
1039,
13,
8094,
3672,
7,
33714,
8,
6624,
12813,
1,
198,
31,
9288,
2546,
7,
33714,
14692,
7785,
8973,
8,
6624,
357,
17,
11,
513,
11,
513,
8,
198,
31,
9288,
2546,
7,
33714,
14692,
7785,
1,
4083,
7785,
8,
6624,
357,
17,
11,
513,
11,
513,
8,
198,
31,
9288,
1438,
7,
33714,
14692,
7785,
1,
4083,
7785,
8,
6624,
366,
7785,
1,
198,
31,
9288,
399,
8610,
265,
292,
1039,
13,
8094,
3672,
7,
33714,
13,
8094,
14692,
1820,
8094,
8973,
8,
6624,
366,
1820,
8094,
1,
628,
198,
2,
2251,
649,
15793,
287,
477,
3696,
198,
33714,
13,
27740,
14692,
3605,
27740,
8973,
796,
17031,
26,
198,
27261,
7,
33714,
1776,
198,
27354,
292,
316,
7,
69,
14933,
58,
16,
12962,
466,
288,
82,
198,
220,
220,
220,
2488,
9288,
288,
82,
13,
27740,
14692,
3605,
27740,
8973,
6624,
17031,
198,
437,
198,
19836,
7,
33714,
8,
198,
46249,
198,
22366,
198
] | 2.107273 | 1,650 |
# Copyright Peter Luschny. License is MIT.
module BasicSwingFactorial
export SwingFactorial
"""
Return the factorial of ``n``. Implementation of the swing algorithm using no
primes. An advanced version based on prime-factorization which is much faster
is available as the prime-swing factorial. However the claim is that this is
the fastest algorithm not using prime-factorization. It has the same recursive
structure as his big brother.
"""
function SwingFactorial(n::Int)::BigInt
smallOddFactorial = BigInt[ 0x0000000000000000000000000000001,
0x0000000000000000000000000000001, 0x0000000000000000000000000000001,
0x0000000000000000000000000000003, 0x0000000000000000000000000000003,
0x000000000000000000000000000000f, 0x000000000000000000000000000002d,
0x000000000000000000000000000013b, 0x000000000000000000000000000013b,
0x0000000000000000000000000000b13, 0x000000000000000000000000000375f,
0x0000000000000000000000000026115, 0x000000000000000000000000007233f,
0x00000000000000000000000005cca33, 0x0000000000000000000000002898765,
0x00000000000000000000000260eeeeb, 0x00000000000000000000000260eeeeb,
0x0000000000000000000000286fddd9b, 0x00000000000000000000016beecca73,
0x000000000000000000001b02b930689, 0x00000000000000000000870d9df20ad,
0x0000000000000000000b141df4dae31, 0x00000000000000000079dd498567c1b,
0x00000000000000000af2e19afc5266d, 0x000000000000000020d8a4d0f4f7347,
0x000000000000000335281867ec241ef, 0x0000000000000029b3093d46fdd5923,
0x0000000000000465e1f9767cc5866b1, 0x0000000000001ec92dd23d6966aced7,
0x0000000000037cca30d0f4f0a196e5b, 0x0000000000344fd8dc3e5a1977d7755,
0x000000000655ab42ab8ce915831734b, 0x000000000655ab42ab8ce915831734b,
0x00000000d10b13981d2a0bc5e5fdcab, 0x0000000de1bc4d19efcac82445da75b,
0x000001e5dcbe8a8bc8b95cf58cde171, 0x00001114c2b2deea0e8444a1f3cecf9,
0x0002780023da37d4191deb683ce3ffd, 0x002ee802a93224bddd3878bc84ebfc7,
0x07255867c6a398ecb39a64b83ff3751, 0x23baba06e131fc9f8203f7993fc1495]
function oddProduct(m, len)
if len < 24
p = BigInt(m)
for k in 2:2:2(len-1)
p *= (m - k)
end
return p
end
hlen = len >> 1
oddProduct(m - 2 * hlen, len - hlen) * oddProduct(m, hlen)
end
function oddFactorial(n)
if n < 41
oddFact = smallOddFactorial[1+n]
sqrOddFact = smallOddFactorial[1+div(n, 2)]
else
sqrOddFact, oldOddFact = oddFactorial(div(n, 2))
len = div(n - 1, 4)
(n % 4) != 2 && (len += 1)
high = n - ((n + 1) & 1)
oddSwing = div(oddProduct(high, len), oldOddFact)
oddFact = sqrOddFact^2 * oddSwing
end
(oddFact, sqrOddFact)
end
n < 0 && ArgumentError("n must be ≥ 0")
if n == 0 return 1 end
sh = n - count_ones(n)
oddFactorial(n)[1] << sh
end
#START-TEST-################################################
using Test
function main()
@testset "SwingFactorial" begin
for n in 0:999
S = SwingFactorial(n)
B = Base.factorial(BigInt(n))
@test S == B
end
end
GC.gc()
n = 1000000
@time SwingFactorial(n)
end
main()
end # module
| [
2,
15069,
5613,
406,
385,
1349,
88,
13,
13789,
318,
17168,
13,
198,
198,
21412,
14392,
50,
5469,
29054,
5132,
198,
39344,
43650,
29054,
5132,
198,
198,
37811,
198,
13615,
262,
1109,
5132,
286,
7559,
77,
15506,
13,
46333,
286,
262,
9628,
11862,
1262,
645,
198,
1050,
999,
13,
1052,
6190,
2196,
1912,
319,
6994,
12,
31412,
1634,
543,
318,
881,
5443,
198,
271,
1695,
355,
262,
6994,
12,
46737,
1109,
5132,
13,
2102,
262,
1624,
318,
326,
428,
318,
220,
198,
1169,
14162,
11862,
407,
1262,
6994,
12,
31412,
1634,
13,
632,
468,
262,
976,
45115,
198,
301,
5620,
355,
465,
1263,
3956,
13,
198,
37811,
198,
8818,
43650,
29054,
5132,
7,
77,
3712,
5317,
2599,
25,
12804,
5317,
628,
220,
220,
220,
1402,
46,
1860,
29054,
5132,
796,
4403,
5317,
58,
220,
220,
220,
220,
220,
220,
220,
657,
87,
25645,
8269,
2388,
8298,
11,
198,
220,
220,
220,
657,
87,
25645,
8269,
2388,
8298,
11,
657,
87,
25645,
8269,
2388,
8298,
11,
198,
220,
220,
220,
657,
87,
25645,
8269,
10535,
18,
11,
657,
87,
25645,
8269,
10535,
18,
11,
198,
220,
220,
220,
657,
87,
25645,
8269,
10535,
69,
11,
657,
87,
25645,
8269,
20483,
17,
67,
11,
198,
220,
220,
220,
657,
87,
25645,
8269,
2388,
1485,
65,
11,
657,
87,
25645,
8269,
2388,
1485,
65,
11,
198,
220,
220,
220,
657,
87,
25645,
8269,
2388,
65,
1485,
11,
657,
87,
25645,
8269,
830,
22318,
69,
11,
198,
220,
220,
220,
657,
87,
25645,
8269,
405,
2075,
15363,
11,
657,
87,
25645,
8269,
25816,
25429,
69,
11,
198,
220,
220,
220,
657,
87,
25645,
10535,
830,
20,
13227,
2091,
11,
657,
87,
25645,
8269,
2078,
4089,
29143,
11,
198,
220,
220,
220,
657,
87,
25645,
24598,
21719,
41591,
65,
11,
657,
87,
25645,
24598,
21719,
41591,
65,
11,
198,
220,
220,
220,
657,
87,
25645,
10535,
27033,
69,
1860,
67,
24,
65,
11,
657,
87,
25645,
2388,
27037,
20963,
13227,
4790,
11,
198,
220,
220,
220,
657,
87,
25645,
2388,
16,
65,
2999,
65,
45418,
40523,
11,
657,
87,
25645,
2388,
46951,
67,
24,
7568,
1238,
324,
11,
198,
220,
220,
220,
657,
87,
25645,
830,
65,
23756,
7568,
19,
67,
3609,
3132,
11,
657,
87,
25645,
405,
3720,
1860,
2920,
5332,
3134,
66,
16,
65,
11,
198,
220,
220,
220,
657,
87,
8269,
10535,
830,
1878,
17,
68,
1129,
1878,
66,
20,
25540,
67,
11,
657,
87,
25645,
1238,
67,
23,
64,
19,
67,
15,
69,
19,
69,
22,
30995,
11,
198,
220,
220,
220,
657,
87,
8269,
24598,
27326,
2078,
1507,
3134,
721,
28872,
891,
11,
657,
87,
8269,
10535,
1959,
65,
1270,
6052,
67,
3510,
69,
1860,
3270,
1954,
11,
198,
220,
220,
220,
657,
87,
8269,
20483,
42018,
68,
16,
69,
5607,
3134,
535,
3365,
2791,
65,
16,
11,
657,
87,
8269,
2388,
16,
721,
5892,
1860,
1954,
67,
3388,
2791,
2286,
22,
11,
198,
220,
220,
220,
657,
87,
8269,
830,
2718,
13227,
1270,
67,
15,
69,
19,
69,
15,
64,
25272,
68,
20,
65,
11,
657,
87,
8269,
11245,
2598,
16344,
23,
17896,
18,
68,
20,
64,
37781,
67,
34483,
20,
11,
198,
220,
220,
220,
657,
87,
10535,
830,
35916,
397,
3682,
397,
23,
344,
40248,
5999,
1558,
2682,
65,
11,
657,
87,
10535,
830,
35916,
397,
3682,
397,
23,
344,
40248,
5999,
1558,
2682,
65,
11,
198,
220,
220,
220,
657,
87,
8269,
67,
940,
65,
1485,
4089,
16,
67,
17,
64,
15,
15630,
20,
68,
20,
16344,
66,
397,
11,
657,
87,
24598,
2934,
16,
15630,
19,
67,
1129,
891,
66,
330,
23,
1731,
2231,
6814,
2425,
65,
11,
198,
220,
220,
220,
657,
87,
2388,
486,
68,
20,
17896,
1350,
23,
64,
23,
15630,
23,
65,
3865,
12993,
3365,
66,
2934,
27192,
11,
657,
87,
2388,
1157,
1415,
66,
17,
65,
17,
67,
1453,
64,
15,
68,
23,
30272,
64,
16,
69,
18,
344,
12993,
24,
11,
198,
220,
220,
220,
657,
87,
830,
1983,
7410,
1954,
6814,
2718,
67,
19,
26492,
11275,
47521,
344,
18,
487,
67,
11,
657,
87,
21601,
1453,
30863,
64,
24,
2624,
1731,
65,
1860,
67,
2548,
3695,
15630,
5705,
1765,
16072,
22,
11,
198,
220,
220,
220,
657,
87,
2998,
1495,
3365,
3134,
66,
21,
64,
31952,
721,
65,
2670,
64,
2414,
65,
5999,
487,
22318,
16,
11,
657,
87,
1954,
65,
15498,
3312,
68,
22042,
16072,
24,
69,
23,
22416,
69,
22,
44821,
16072,
1415,
3865,
60,
628,
220,
220,
220,
2163,
5629,
15667,
7,
76,
11,
18896,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
18896,
1279,
1987,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
279,
796,
4403,
5317,
7,
76,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
329,
479,
287,
362,
25,
17,
25,
17,
7,
11925,
12,
16,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
279,
1635,
28,
357,
76,
532,
479,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1441,
279,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
289,
11925,
796,
18896,
9609,
352,
198,
220,
220,
220,
220,
220,
220,
220,
5629,
15667,
7,
76,
532,
362,
1635,
289,
11925,
11,
18896,
532,
289,
11925,
8,
1635,
5629,
15667,
7,
76,
11,
289,
11925,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
2163,
5629,
29054,
5132,
7,
77,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
299,
1279,
6073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
5629,
29054,
796,
1402,
46,
1860,
29054,
5132,
58,
16,
10,
77,
60,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
19862,
81,
46,
1860,
29054,
796,
1402,
46,
1860,
29054,
5132,
58,
16,
10,
7146,
7,
77,
11,
362,
15437,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
19862,
81,
46,
1860,
29054,
11,
1468,
46,
1860,
29054,
796,
5629,
29054,
5132,
7,
7146,
7,
77,
11,
362,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
18896,
796,
2659,
7,
77,
532,
352,
11,
604,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
357,
77,
4064,
604,
8,
14512,
362,
11405,
357,
11925,
15853,
352,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1029,
796,
299,
532,
14808,
77,
1343,
352,
8,
1222,
352,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
5629,
50,
5469,
796,
2659,
7,
5088,
15667,
7,
8929,
11,
18896,
828,
1468,
46,
1860,
29054,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
5629,
29054,
796,
19862,
81,
46,
1860,
29054,
61,
17,
1635,
5629,
50,
5469,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
357,
5088,
29054,
11,
19862,
81,
46,
1860,
29054,
8,
198,
220,
220,
220,
886,
628,
220,
220,
220,
299,
1279,
657,
11405,
45751,
12331,
7203,
77,
1276,
307,
26870,
657,
4943,
198,
220,
220,
220,
611,
299,
6624,
657,
1441,
352,
886,
198,
220,
220,
220,
427,
796,
299,
532,
954,
62,
1952,
7,
77,
8,
198,
220,
220,
220,
5629,
29054,
5132,
7,
77,
38381,
16,
60,
9959,
427,
198,
437,
198,
198,
2,
2257,
7227,
12,
51,
6465,
12,
29113,
14468,
198,
3500,
6208,
198,
198,
8818,
1388,
3419,
198,
220,
220,
220,
2488,
9288,
2617,
366,
50,
5469,
29054,
5132,
1,
2221,
198,
220,
220,
220,
220,
220,
220,
220,
329,
299,
287,
657,
25,
17032,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
311,
796,
43650,
29054,
5132,
7,
77,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
347,
796,
7308,
13,
22584,
5132,
7,
12804,
5317,
7,
77,
4008,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
9288,
311,
6624,
347,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
20145,
13,
36484,
3419,
198,
220,
220,
220,
299,
796,
1802,
2388,
198,
220,
220,
220,
2488,
2435,
43650,
29054,
5132,
7,
77,
8,
198,
437,
198,
198,
12417,
3419,
198,
198,
437,
1303,
8265,
198
] | 2.3284 | 1,419 |
#This script calculates the squeezing for different quadratures.
using LinearAlgebra
using Plots; pyplot()
using NPZ
using LaTeXStrings
include("MagnusExpansion.jl")
include("boson.jl")
include("HarmonicCorrectionBoson.jl")
"""
Calculate the uncertainties on the x(θ) and y(θ) quadratures for
a range imax values of θ in the interval [θi, θi+θrange].
"""
function quadrature_scan(moments, imax; θi=-pi/4, θrange=pi/2)
θ_vec, Δx2_vec, Δy2_vec = zeros(imax), zeros(imax), zeros(imax)
for i = 1: imax
θ = θi + (i - 1)*θrange/(imax - 1)
θ_vec[i] = θ
Δx2_vec[i] = (moments[3] + cos(2*θ)*moments[1] + sin(2*θ)*moments[2])/2
Δy2_vec[i] = (moments[3] - cos(2*θ)*moments[1] - sin(2*θ)*moments[2])/2
end
return θ_vec, Δx2_vec, Δy2_vec
end
"""
Apply the function quadrature_scan a couple of times, always around the value of θ
where maximum squeezing has been found.
"""
function fine_quadrature_scan(moments, imax)
θ_vec, Δx2_vec, Δy2_vec = quadrature_scan(moments, imax)
for i = 1: 4
k = argmin(Δy2_vec)
θi = θ_vec[k - 1]
θrange = θ_vec[k + 1] - θ_vec[k - 1]
output = quadrature_scan(moments, imax; θi=θi, θrange=θrange)
θ_vec .= output[1]
Δx2_vec .= output[2]
Δy2_vec .= output[3]
end
return θ_vec, Δx2_vec, Δy2_vec
end
#array with different gate times tf
tf0, tf1 = 2.0, 15.0
nt = 200
tf_vec = zeros(nt)
nt = size(tf_vec)[1]
dB_vec = zeros(nt, 3)
moments = zeros(nt, 3)
coeffs_array = zeros(nt, 3)
imax = 10
θopt = zeros(nt,2)
for j = 1: nt
println(j)
tf = tf0*(tf1/tf0)^((j - 1)/(nt - 1))
tf_vec[j] = tf
κ = 0.0
# solution in the absence of counter-rotating terms
cr_terms = false
moments[j, :] = solve_2moments(tf, κ, cr_terms, zeros(3))
Δx2 = (moments[j, 3] + moments[j, 1])/2
Δy2 = (moments[j, 3] - moments[j, 1])/2
dB_vec[j, 1] = -10.0*log10(2*Δy2)
# solution in the presence of counter-rotating terms
cr_terms = true
moments[j, :] = solve_2moments(tf, κ, cr_terms, zeros(3))
Δx2 = (moments[j, 3] + moments[j, 1])/2
Δy2 = (moments[j, 3] - moments[j, 1])/2
dB_vec[j, 2] = -10.0*log10(2*Δy2)
θ_vec, Δx2_vec, Δy2_vec = fine_quadrature_scan(moments[j, :], imax)
k = argmin(Δy2_vec)
θopt[j, 1] = θ_vec[k]
# solution in the presence of counter-rotating terms and correction.
cr_terms = true
M = correction_matrix(tf)
order = 6
coeffs = get_coeffs(M, order, tf)
coeffs_array[j, :] = coeffs
moments[j, :] = solve_2moments(tf, κ, cr_terms, coeffs)
Δx2 = (moments[j, 3] + moments[j, 1])/2
Δy2 = (moments[j, 3] - moments[j, 1])/2
dB_vec[j, 3] = -10.0*log10(2*Δy2)
θ_vec, Δx2_vec, Δy2_vec = fine_quadrature_scan(moments[j, :], imax)
k = argmin(Δy2_vec)
θopt[j, 2] = θ_vec[k]
end
# save figures
plot(tf_vec, dB_vec,
linewidth = 2,
labels = ["No correction" "6th order\nMagnus" "RWA"])
plot!(tickfont = font(12, "Serif"),
xlabel = "Pulse width "*L"\omega_\mathrm{a} t_\mathrm{f}",
ylabel = "Squeezing (dB)",
guidefont = font(14, "Serif"),
legendfont = font(12, "Serif"),
background_color_legend = false,
margin = 5Plots.mm)
savefig("squeezing.pdf")
y = hcat(θopt, zeros(size(θopt)[1]))
plot(tf_vec, y,
linewidth = 2,
labels = ["No correction" "6th order\nMagnus" "RWA"])
plot!(tickfont = font(12, "Serif"),
xlabel = "Pulse width "*L"\omega_\mathrm{a} t_\mathrm{f}",
ylabel = L"\varphi",
guidefont = font(14, "Serif"),
legendfont = font(12, "Serif"),
background_color_legend = false,
margin = 5Plots.mm)
savefig("squeezing_angle.pdf")
y = hcat(1.0./tf_vec, abs.(coeffs_array))
plot(tf_vec, y,
linewidth = 2,
labels = [L"1/t_\mathrm{f}" L"|c_{x,1}|" L"|c_{y,1}|" L"|\Delta|"])
plot!(yaxis = :log,
tickfont = font(12, "Serif"),
xlabel = "Pulse width "*L"\omega_\mathrm{a} t_\mathrm{f}",
ylabel = "coeff."*L"\times \omega_\mathrm{a}^{-1}",
guidefont = font(14, "Serif"),
legendfont = font(12, "Serif"),
background_color_legend = false,
margin = 5Plots.mm)
savefig("coeffs.pdf")
# save data files
output = zeros(nt,4)
output[:, 1] = tf_vec
output[:, 2: end] = dB_vec
npzwrite("dB.npy", output)
output = zeros(nt,3)
output[:, 1] = tf_vec
output[:, 2: end] = θopt
npzwrite("angle.npy", output)
output = zeros(nt,4)
output[:, 1] = tf_vec
output[:, 2: end] = coeffs_array
npzwrite("coeffs.npy", output)
| [
2,
1212,
4226,
43707,
262,
40237,
329,
1180,
15094,
81,
6691,
13,
198,
3500,
44800,
2348,
29230,
198,
3500,
1345,
1747,
26,
12972,
29487,
3419,
198,
3500,
28498,
57,
198,
3500,
4689,
49568,
13290,
654,
198,
198,
17256,
7203,
48017,
385,
16870,
5487,
13,
20362,
4943,
198,
17256,
7203,
39565,
261,
13,
20362,
4943,
198,
17256,
7203,
39,
1670,
9229,
43267,
33,
418,
261,
13,
20362,
4943,
198,
198,
37811,
198,
9771,
3129,
378,
262,
36553,
319,
262,
2124,
7,
138,
116,
8,
290,
331,
7,
138,
116,
8,
15094,
81,
6691,
329,
198,
64,
2837,
545,
897,
3815,
286,
7377,
116,
287,
262,
16654,
685,
138,
116,
72,
11,
7377,
116,
72,
10,
138,
116,
9521,
4083,
198,
37811,
198,
8818,
15094,
81,
1300,
62,
35836,
7,
32542,
658,
11,
545,
897,
26,
7377,
116,
72,
10779,
14415,
14,
19,
11,
7377,
116,
9521,
28,
14415,
14,
17,
8,
198,
197,
138,
116,
62,
35138,
11,
37455,
87,
17,
62,
35138,
11,
37455,
88,
17,
62,
35138,
796,
1976,
27498,
7,
320,
897,
828,
1976,
27498,
7,
320,
897,
828,
1976,
27498,
7,
320,
897,
8,
198,
197,
1640,
1312,
796,
352,
25,
545,
897,
198,
197,
197,
138,
116,
796,
7377,
116,
72,
1343,
357,
72,
532,
352,
27493,
138,
116,
9521,
29006,
320,
897,
532,
352,
8,
198,
197,
197,
138,
116,
62,
35138,
58,
72,
60,
796,
7377,
116,
198,
197,
197,
138,
242,
87,
17,
62,
35138,
58,
72,
60,
796,
357,
32542,
658,
58,
18,
60,
1343,
8615,
7,
17,
9,
138,
116,
27493,
32542,
658,
58,
16,
60,
1343,
7813,
7,
17,
9,
138,
116,
27493,
32542,
658,
58,
17,
12962,
14,
17,
198,
197,
197,
138,
242,
88,
17,
62,
35138,
58,
72,
60,
796,
357,
32542,
658,
58,
18,
60,
532,
8615,
7,
17,
9,
138,
116,
27493,
32542,
658,
58,
16,
60,
532,
7813,
7,
17,
9,
138,
116,
27493,
32542,
658,
58,
17,
12962,
14,
17,
198,
197,
437,
198,
197,
7783,
7377,
116,
62,
35138,
11,
37455,
87,
17,
62,
35138,
11,
37455,
88,
17,
62,
35138,
198,
437,
198,
37811,
198,
44836,
262,
2163,
15094,
81,
1300,
62,
35836,
257,
3155,
286,
1661,
11,
1464,
1088,
262,
1988,
286,
7377,
116,
198,
3003,
5415,
40237,
468,
587,
1043,
13,
198,
37811,
198,
8818,
3734,
62,
421,
41909,
1300,
62,
35836,
7,
32542,
658,
11,
545,
897,
8,
198,
197,
138,
116,
62,
35138,
11,
37455,
87,
17,
62,
35138,
11,
37455,
88,
17,
62,
35138,
796,
15094,
81,
1300,
62,
35836,
7,
32542,
658,
11,
545,
897,
8,
198,
197,
1640,
1312,
796,
352,
25,
604,
198,
197,
197,
74,
796,
1822,
1084,
7,
138,
242,
88,
17,
62,
35138,
8,
198,
197,
197,
138,
116,
72,
796,
7377,
116,
62,
35138,
58,
74,
532,
352,
60,
198,
197,
197,
138,
116,
9521,
796,
7377,
116,
62,
35138,
58,
74,
1343,
352,
60,
532,
7377,
116,
62,
35138,
58,
74,
532,
352,
60,
198,
197,
197,
22915,
796,
15094,
81,
1300,
62,
35836,
7,
32542,
658,
11,
545,
897,
26,
7377,
116,
72,
28,
138,
116,
72,
11,
7377,
116,
9521,
28,
138,
116,
9521,
8,
198,
197,
197,
138,
116,
62,
35138,
764,
28,
5072,
58,
16,
60,
198,
197,
197,
138,
242,
87,
17,
62,
35138,
764,
28,
5072,
58,
17,
60,
198,
197,
197,
138,
242,
88,
17,
62,
35138,
764,
28,
5072,
58,
18,
60,
198,
197,
437,
198,
197,
7783,
7377,
116,
62,
35138,
11,
37455,
87,
17,
62,
35138,
11,
37455,
88,
17,
62,
35138,
198,
437,
198,
198,
2,
18747,
351,
1180,
8946,
1661,
48700,
198,
27110,
15,
11,
48700,
16,
796,
362,
13,
15,
11,
1315,
13,
15,
198,
429,
796,
939,
198,
27110,
62,
35138,
796,
1976,
27498,
7,
429,
8,
198,
429,
796,
2546,
7,
27110,
62,
35138,
38381,
16,
60,
198,
36077,
62,
35138,
796,
1976,
27498,
7,
429,
11,
513,
8,
198,
32542,
658,
796,
1976,
27498,
7,
429,
11,
513,
8,
198,
1073,
14822,
82,
62,
18747,
796,
1976,
27498,
7,
429,
11,
513,
8,
198,
198,
320,
897,
796,
838,
198,
138,
116,
8738,
796,
1976,
27498,
7,
429,
11,
17,
8,
198,
198,
1640,
474,
796,
352,
25,
299,
83,
198,
197,
35235,
7,
73,
8,
198,
197,
27110,
796,
48700,
15,
9,
7,
27110,
16,
14,
27110,
15,
8,
61,
19510,
73,
532,
352,
20679,
7,
429,
532,
352,
4008,
198,
197,
27110,
62,
35138,
58,
73,
60,
796,
48700,
198,
197,
43000,
796,
657,
13,
15,
198,
197,
2,
4610,
287,
262,
8889,
286,
3753,
12,
10599,
803,
2846,
198,
197,
6098,
62,
38707,
796,
3991,
198,
197,
32542,
658,
58,
73,
11,
1058,
60,
796,
8494,
62,
17,
32542,
658,
7,
27110,
11,
7377,
118,
11,
1067,
62,
38707,
11,
1976,
27498,
7,
18,
4008,
198,
197,
138,
242,
87,
17,
796,
357,
32542,
658,
58,
73,
11,
513,
60,
1343,
7188,
58,
73,
11,
352,
12962,
14,
17,
198,
197,
138,
242,
88,
17,
796,
357,
32542,
658,
58,
73,
11,
513,
60,
532,
7188,
58,
73,
11,
352,
12962,
14,
17,
198,
197,
36077,
62,
35138,
58,
73,
11,
352,
60,
796,
532,
940,
13,
15,
9,
6404,
940,
7,
17,
9,
138,
242,
88,
17,
8,
628,
197,
2,
4610,
287,
262,
4931,
286,
3753,
12,
10599,
803,
2846,
198,
197,
6098,
62,
38707,
796,
2081,
198,
197,
32542,
658,
58,
73,
11,
1058,
60,
796,
8494,
62,
17,
32542,
658,
7,
27110,
11,
7377,
118,
11,
1067,
62,
38707,
11,
1976,
27498,
7,
18,
4008,
198,
197,
138,
242,
87,
17,
796,
357,
32542,
658,
58,
73,
11,
513,
60,
1343,
7188,
58,
73,
11,
352,
12962,
14,
17,
198,
197,
138,
242,
88,
17,
796,
357,
32542,
658,
58,
73,
11,
513,
60,
532,
7188,
58,
73,
11,
352,
12962,
14,
17,
198,
197,
36077,
62,
35138,
58,
73,
11,
362,
60,
796,
532,
940,
13,
15,
9,
6404,
940,
7,
17,
9,
138,
242,
88,
17,
8,
628,
197,
138,
116,
62,
35138,
11,
37455,
87,
17,
62,
35138,
11,
37455,
88,
17,
62,
35138,
796,
3734,
62,
421,
41909,
1300,
62,
35836,
7,
32542,
658,
58,
73,
11,
1058,
4357,
545,
897,
8,
198,
197,
74,
796,
1822,
1084,
7,
138,
242,
88,
17,
62,
35138,
8,
198,
197,
138,
116,
8738,
58,
73,
11,
352,
60,
796,
7377,
116,
62,
35138,
58,
74,
60,
628,
197,
2,
4610,
287,
262,
4931,
286,
3753,
12,
10599,
803,
2846,
290,
17137,
13,
198,
197,
6098,
62,
38707,
796,
2081,
198,
197,
44,
796,
17137,
62,
6759,
8609,
7,
27110,
8,
198,
197,
2875,
796,
718,
198,
197,
1073,
14822,
82,
796,
651,
62,
1073,
14822,
82,
7,
44,
11,
1502,
11,
48700,
8,
628,
197,
1073,
14822,
82,
62,
18747,
58,
73,
11,
1058,
60,
796,
763,
14822,
82,
198,
197,
32542,
658,
58,
73,
11,
1058,
60,
796,
8494,
62,
17,
32542,
658,
7,
27110,
11,
7377,
118,
11,
1067,
62,
38707,
11,
763,
14822,
82,
8,
198,
197,
138,
242,
87,
17,
796,
357,
32542,
658,
58,
73,
11,
513,
60,
1343,
7188,
58,
73,
11,
352,
12962,
14,
17,
198,
197,
138,
242,
88,
17,
796,
357,
32542,
658,
58,
73,
11,
513,
60,
532,
7188,
58,
73,
11,
352,
12962,
14,
17,
198,
197,
36077,
62,
35138,
58,
73,
11,
513,
60,
796,
532,
940,
13,
15,
9,
6404,
940,
7,
17,
9,
138,
242,
88,
17,
8,
628,
197,
138,
116,
62,
35138,
11,
37455,
87,
17,
62,
35138,
11,
37455,
88,
17,
62,
35138,
796,
3734,
62,
421,
41909,
1300,
62,
35836,
7,
32542,
658,
58,
73,
11,
1058,
4357,
545,
897,
8,
198,
197,
74,
796,
1822,
1084,
7,
138,
242,
88,
17,
62,
35138,
8,
198,
197,
138,
116,
8738,
58,
73,
11,
362,
60,
796,
7377,
116,
62,
35138,
58,
74,
60,
198,
437,
198,
2,
3613,
5538,
198,
29487,
7,
27110,
62,
35138,
11,
30221,
62,
35138,
11,
198,
197,
9493,
413,
5649,
796,
362,
11,
198,
197,
14722,
796,
14631,
2949,
17137,
1,
366,
21,
400,
1502,
59,
77,
48017,
385,
1,
366,
49,
15543,
8973,
8,
198,
29487,
0,
7,
42298,
10331,
796,
10369,
7,
1065,
11,
366,
7089,
361,
12340,
198,
197,
220,
2124,
18242,
796,
366,
47,
9615,
9647,
366,
9,
43,
1,
59,
462,
4908,
62,
59,
11018,
26224,
90,
64,
92,
256,
62,
59,
11018,
26224,
90,
69,
92,
1600,
198,
197,
220,
331,
18242,
796,
366,
22266,
1453,
9510,
357,
36077,
42501,
198,
197,
220,
5698,
10331,
796,
10369,
7,
1415,
11,
366,
7089,
361,
12340,
198,
197,
220,
8177,
10331,
796,
10369,
7,
1065,
11,
366,
7089,
361,
12340,
198,
197,
220,
4469,
62,
8043,
62,
1455,
437,
796,
3991,
11,
198,
197,
220,
10330,
796,
642,
3646,
1747,
13,
3020,
8,
198,
21928,
5647,
7203,
16485,
1453,
9510,
13,
12315,
4943,
198,
198,
88,
796,
289,
9246,
7,
138,
116,
8738,
11,
1976,
27498,
7,
7857,
7,
138,
116,
8738,
38381,
16,
60,
4008,
198,
29487,
7,
27110,
62,
35138,
11,
331,
11,
198,
197,
9493,
413,
5649,
796,
362,
11,
198,
197,
14722,
796,
14631,
2949,
17137,
1,
366,
21,
400,
1502,
59,
77,
48017,
385,
1,
366,
49,
15543,
8973,
8,
198,
29487,
0,
7,
42298,
10331,
796,
10369,
7,
1065,
11,
366,
7089,
361,
12340,
198,
197,
220,
2124,
18242,
796,
366,
47,
9615,
9647,
366,
9,
43,
1,
59,
462,
4908,
62,
59,
11018,
26224,
90,
64,
92,
256,
62,
59,
11018,
26224,
90,
69,
92,
1600,
198,
197,
220,
331,
18242,
796,
406,
1,
59,
7785,
34846,
1600,
198,
197,
220,
5698,
10331,
796,
10369,
7,
1415,
11,
366,
7089,
361,
12340,
198,
197,
220,
8177,
10331,
796,
10369,
7,
1065,
11,
366,
7089,
361,
12340,
198,
197,
220,
4469,
62,
8043,
62,
1455,
437,
796,
3991,
11,
198,
197,
220,
10330,
796,
642,
3646,
1747,
13,
3020,
8,
198,
21928,
5647,
7203,
16485,
1453,
9510,
62,
9248,
13,
12315,
4943,
198,
198,
88,
796,
289,
9246,
7,
16,
13,
15,
19571,
27110,
62,
35138,
11,
2352,
12195,
1073,
14822,
82,
62,
18747,
4008,
198,
29487,
7,
27110,
62,
35138,
11,
331,
11,
198,
197,
9493,
413,
5649,
796,
362,
11,
198,
197,
14722,
796,
685,
43,
1,
16,
14,
83,
62,
59,
11018,
26224,
90,
69,
36786,
406,
1,
91,
66,
23330,
87,
11,
16,
92,
91,
1,
406,
1,
91,
66,
23330,
88,
11,
16,
92,
91,
1,
406,
1,
91,
59,
42430,
91,
8973,
8,
198,
29487,
0,
7,
88,
22704,
796,
1058,
6404,
11,
198,
197,
220,
4378,
10331,
796,
10369,
7,
1065,
11,
366,
7089,
361,
12340,
198,
197,
220,
2124,
18242,
796,
366,
47,
9615,
9647,
366,
9,
43,
1,
59,
462,
4908,
62,
59,
11018,
26224,
90,
64,
92,
256,
62,
59,
11018,
26224,
90,
69,
92,
1600,
198,
197,
220,
331,
18242,
796,
366,
1073,
14822,
526,
9,
43,
1,
59,
22355,
3467,
462,
4908,
62,
59,
11018,
26224,
90,
64,
92,
36796,
12,
16,
92,
1600,
198,
197,
220,
5698,
10331,
796,
10369,
7,
1415,
11,
366,
7089,
361,
12340,
198,
197,
220,
8177,
10331,
796,
10369,
7,
1065,
11,
366,
7089,
361,
12340,
198,
197,
220,
4469,
62,
8043,
62,
1455,
437,
796,
3991,
11,
198,
197,
220,
10330,
796,
642,
3646,
1747,
13,
3020,
8,
198,
21928,
5647,
7203,
1073,
14822,
82,
13,
12315,
4943,
198,
198,
2,
3613,
1366,
3696,
198,
22915,
796,
1976,
27498,
7,
429,
11,
19,
8,
198,
22915,
58,
45299,
352,
60,
796,
48700,
62,
35138,
198,
22915,
58,
45299,
362,
25,
886,
60,
796,
30221,
62,
35138,
198,
37659,
89,
13564,
7203,
36077,
13,
77,
9078,
1600,
5072,
8,
198,
22915,
796,
1976,
27498,
7,
429,
11,
18,
8,
198,
22915,
58,
45299,
352,
60,
796,
48700,
62,
35138,
198,
22915,
58,
45299,
362,
25,
886,
60,
796,
7377,
116,
8738,
198,
37659,
89,
13564,
7203,
9248,
13,
77,
9078,
1600,
5072,
8,
198,
22915,
796,
1976,
27498,
7,
429,
11,
19,
8,
198,
22915,
58,
45299,
352,
60,
796,
48700,
62,
35138,
198,
22915,
58,
45299,
362,
25,
886,
60,
796,
763,
14822,
82,
62,
18747,
198,
37659,
89,
13564,
7203,
1073,
14822,
82,
13,
77,
9078,
1600,
5072,
8,
198
] | 2.059512 | 2,050 |
using LinearAlgebra, SparseArrays, Test
using MultivariatePolynomials
using SumOfSquares
# Taken from JuMP/test/solvers.jl
function try_import(name::Symbol)
try
@eval import $name
return true
catch e
return false
end
end
if try_import(:DynamicPolynomials)
import DynamicPolynomials.@polyvar
import DynamicPolynomials.@ncpolyvar
else
if try_import(:TypedPolynomials)
import TypedPolynomials.@polyvar
else
error("No polynomial implementation installed : Please install TypedPolynomials or DynamicPolynomials")
end
end
include("certificate.jl")
include("gram_matrix.jl")
include("variable.jl")
include("constraint.jl")
include("Mock/mock_tests.jl")
# Tests needing a solver
# FIXME these tests should be converted to Literate and moved to `examples` or
# converted to be used with `MockOptimizer` and moved to `test/Tests`
include("solvers.jl")
include("sospoly.jl")
include("sosquartic.jl")
include("equalitypolyconstr.jl")
| [
3500,
44800,
2348,
29230,
11,
1338,
17208,
3163,
20477,
11,
6208,
198,
198,
3500,
7854,
42524,
34220,
26601,
8231,
198,
3500,
5060,
5189,
22266,
3565,
198,
198,
2,
30222,
422,
12585,
7378,
14,
9288,
14,
34453,
690,
13,
20362,
198,
8818,
1949,
62,
11748,
7,
3672,
3712,
13940,
23650,
8,
198,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
18206,
1330,
720,
3672,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
2081,
198,
220,
220,
220,
4929,
304,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
3991,
198,
220,
220,
220,
886,
198,
437,
198,
198,
361,
1949,
62,
11748,
7,
25,
44090,
34220,
26601,
8231,
8,
198,
220,
220,
220,
1330,
26977,
34220,
26601,
8231,
13,
31,
35428,
7785,
198,
220,
220,
220,
1330,
26977,
34220,
26601,
8231,
13,
31,
10782,
35428,
7785,
198,
17772,
198,
220,
220,
220,
611,
1949,
62,
11748,
7,
25,
31467,
276,
34220,
26601,
8231,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1330,
17134,
276,
34220,
26601,
8231,
13,
31,
35428,
7785,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
4049,
7203,
2949,
745,
6213,
49070,
7822,
6589,
1058,
4222,
2721,
17134,
276,
34220,
26601,
8231,
393,
26977,
34220,
26601,
8231,
4943,
198,
220,
220,
220,
886,
198,
437,
198,
198,
17256,
7203,
22583,
22460,
13,
20362,
4943,
198,
198,
17256,
7203,
4546,
62,
6759,
8609,
13,
20362,
4943,
198,
198,
17256,
7203,
45286,
13,
20362,
4943,
198,
17256,
7203,
1102,
2536,
2913,
13,
20362,
4943,
198,
198,
17256,
7203,
44,
735,
14,
76,
735,
62,
41989,
13,
20362,
4943,
198,
198,
2,
30307,
18139,
257,
1540,
332,
198,
2,
44855,
11682,
777,
5254,
815,
307,
11513,
284,
17667,
378,
290,
3888,
284,
4600,
1069,
12629,
63,
393,
198,
2,
11513,
284,
307,
973,
351,
4600,
44,
735,
27871,
320,
7509,
63,
290,
3888,
284,
4600,
9288,
14,
51,
3558,
63,
198,
17256,
7203,
34453,
690,
13,
20362,
4943,
198,
17256,
7203,
82,
2117,
3366,
13,
20362,
4943,
198,
17256,
7203,
82,
418,
36008,
291,
13,
20362,
4943,
198,
17256,
7203,
48203,
35428,
1102,
2536,
13,
20362,
4943,
198
] | 2.791086 | 359 |
# ------------------------------------------------------------------
# Licensed under the MIT License. See LICENSE in the project root.
# ------------------------------------------------------------------
"""
DensityRatioWeighting(tdata, [vars]; [options])
Density ratio weights based on empirical distribution of
variables in target data `tdata`. Default to all variables.
## Optional parameters
* `estimator` - Density ratio estimator (default to `LSIF()`)
* `optlib` - Optimization library (default to `default_optlib(estimator)`)
### Notes
Estimators from `DensityRatioEstimation.jl` are supported.
"""
struct DensityRatioWeighting <: WeightingMethod
tdata
vars
dre
optlib
end
function DensityRatioWeighting(tdata, vars=nothing; estimator=LSIF(),
optlib=default_optlib(estimator))
validvars = collect(name.(variables(tdata)))
wvars = isnothing(vars) ? validvars : vars
@assert wvars ⊆ validvars "invalid variables ($wvars) for spatial data"
DensityRatioWeighting(tdata, wvars, estimator, optlib)
end
function weight(sdata, method::DensityRatioWeighting)
# retrieve method parameters
tdata = method.tdata
vars = method.vars
dre = method.dre
optlib = method.optlib
@assert vars ⊆ name.(variables(sdata)) "invalid variables ($vars) for spatial data"
# numerator and denominator samples
Ωnu = view(tdata, vars)
Ωde = view(sdata, vars)
xnu = collect(Tables.rows(values(Ωnu)))
xde = collect(Tables.rows(values(Ωde)))
# perform denstiy ratio estimation
ratios = densratio(xnu, xde, dre, optlib=optlib)
SpatialWeights(domain(sdata), ratios)
end
| [
2,
16529,
438,
198,
2,
49962,
739,
262,
17168,
13789,
13,
4091,
38559,
24290,
287,
262,
1628,
6808,
13,
198,
2,
16529,
438,
198,
198,
37811,
198,
220,
220,
220,
360,
6377,
29665,
952,
25844,
278,
7,
83,
7890,
11,
685,
85,
945,
11208,
685,
25811,
12962,
198,
198,
35,
6377,
8064,
19590,
1912,
319,
21594,
6082,
286,
198,
25641,
2977,
287,
2496,
1366,
4600,
83,
7890,
44646,
15161,
284,
477,
9633,
13,
198,
198,
2235,
32233,
10007,
198,
198,
9,
4600,
395,
320,
1352,
63,
532,
360,
6377,
8064,
3959,
1352,
357,
12286,
284,
4600,
6561,
5064,
3419,
63,
8,
198,
9,
4600,
8738,
8019,
63,
220,
220,
220,
532,
30011,
1634,
5888,
357,
12286,
284,
4600,
12286,
62,
8738,
8019,
7,
395,
320,
1352,
8,
63,
8,
198,
198,
21017,
11822,
198,
198,
22362,
320,
2024,
422,
4600,
35,
6377,
29665,
952,
22362,
18991,
13,
20362,
63,
389,
4855,
13,
198,
37811,
198,
7249,
360,
6377,
29665,
952,
25844,
278,
1279,
25,
14331,
278,
17410,
198,
220,
256,
7890,
198,
220,
410,
945,
198,
220,
288,
260,
198,
220,
2172,
8019,
198,
437,
198,
198,
8818,
360,
6377,
29665,
952,
25844,
278,
7,
83,
7890,
11,
410,
945,
28,
22366,
26,
3959,
1352,
28,
6561,
5064,
22784,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2172,
8019,
28,
12286,
62,
8738,
8019,
7,
395,
320,
1352,
4008,
198,
220,
4938,
85,
945,
796,
2824,
7,
3672,
12195,
25641,
2977,
7,
83,
7890,
22305,
198,
220,
266,
85,
945,
796,
318,
22366,
7,
85,
945,
8,
5633,
4938,
85,
945,
1058,
410,
945,
198,
220,
2488,
30493,
266,
85,
945,
2343,
232,
228,
4938,
85,
945,
366,
259,
12102,
9633,
7198,
86,
85,
945,
8,
329,
21739,
1366,
1,
198,
220,
360,
6377,
29665,
952,
25844,
278,
7,
83,
7890,
11,
266,
85,
945,
11,
3959,
1352,
11,
2172,
8019,
8,
198,
437,
198,
198,
8818,
3463,
7,
82,
7890,
11,
2446,
3712,
35,
6377,
29665,
952,
25844,
278,
8,
198,
220,
1303,
19818,
2446,
10007,
198,
220,
256,
7890,
220,
796,
2446,
13,
83,
7890,
198,
220,
410,
945,
220,
220,
796,
2446,
13,
85,
945,
198,
220,
288,
260,
220,
220,
220,
796,
2446,
13,
67,
260,
198,
220,
2172,
8019,
796,
2446,
13,
8738,
8019,
628,
220,
2488,
30493,
410,
945,
2343,
232,
228,
1438,
12195,
25641,
2977,
7,
82,
7890,
4008,
366,
259,
12102,
9633,
7198,
85,
945,
8,
329,
21739,
1366,
1,
628,
220,
1303,
5470,
1352,
290,
31457,
1352,
8405,
198,
220,
7377,
102,
28803,
796,
1570,
7,
83,
7890,
11,
410,
945,
8,
198,
220,
7377,
102,
2934,
796,
1570,
7,
82,
7890,
11,
410,
945,
8,
198,
220,
2124,
28803,
796,
2824,
7,
51,
2977,
13,
8516,
7,
27160,
7,
138,
102,
28803,
22305,
198,
220,
2124,
2934,
796,
2824,
7,
51,
2977,
13,
8516,
7,
27160,
7,
138,
102,
2934,
22305,
628,
220,
1303,
1620,
2853,
301,
7745,
8064,
31850,
198,
220,
22423,
796,
29509,
10366,
952,
7,
87,
28803,
11,
2124,
2934,
11,
288,
260,
11,
2172,
8019,
28,
8738,
8019,
8,
628,
220,
1338,
34961,
1135,
2337,
7,
27830,
7,
82,
7890,
828,
22423,
8,
198,
437,
198
] | 2.96745 | 553 |
function eval_ast(interpstate::InterpState, mod, expr)
interpstate.options.debug && @show :eval_ast mod expr
try
# ans = Core.eval(mod, expr)
ans = @eval mod $expr
interpstate.options.debug && @show :eval_ast ans
return ans
catch exception
interpstate.options.debug && @show :eval_ast mod expr exception
rethrow()
end
end
function eval_lower_ast(interpstate::InterpState, mod, expr)
interpstate.options.debug && @show :eval_lower_ast mod expr
try
lwr = Meta.lower(mod, expr)::Expr
if lwr.head == :thunk
codestate = code_state_from_thunk(interpstate, mod, lwr.args[1]::Core.CodeInfo)
ans = interpret_lower(codestate)
else
@show lwr mod expr
# ans = Core.eval(mod, expr)
ans = @eval mod $expr
end
interpstate.options.debug && @show :eval_lower_ast ans
return ans
catch exception
interpstate.options.debug && @show :eval_lower_ast mod expr exception
rethrow()
end
end
function interpret_ast_comparison(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :comparison args
expr = Expr(:comparison, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_or(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :(||) args
expr = Expr(:(||), args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_and(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :(&&) args
expr = Expr(:(&&), args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_primitive(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :primitive args
expr = Expr(:primitive, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_call(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :call args
expr = Expr(:call, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_macrocall(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :macrocall args
expr = Expr(:macrocall, args...)
# Macros be better handled by the compiler?
eval_ast(interpstate, mod, expr)
end
function interpret_ast_broadcast(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :(.=) args
expr = Expr(:(.=), args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_assign(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :(=) args
expr = Expr(:(=), args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_const(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :const args
expr = Expr(:const, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_local(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :local args
expr = Expr(:local, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_global(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :global args
expr = Expr(:global, args...)
eval_ast(interpstate, mod, expr)
end
function interpret_ast_let(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :let args
expr = Expr(:let, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_block(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :block args
local linenumbernode
try
local ans
for arg in args
ans = interpret_ast_node(interpstate, mod, arg)
if ans isa LineNumberNode
linenumbernode = ans
end
end
ans
catch
@show linenumbernode
rethrow()
end
end
function interpret_ast_try(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :try args
expr = Expr(:try, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_do(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :do args
expr = Expr(:do, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_if(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :if args
expr = Expr(:if, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_for(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :for args
expr = Expr(:for, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_while(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :while args
expr = Expr(:while, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_function(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :function args
expr = Expr(:function, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_where(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :function args
expr = Expr(:where, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_macro(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :macro args
expr = Expr(:macro, args...)
# Macros be better handled by the compiler?
eval_ast(interpstate, mod, expr)
end
function interpret_ast_struct(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :struct args
expr = Expr(:struct, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_curly(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :curly args
expr = Expr(:curly, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_abstract(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :abstract args
expr = Expr(:abstract, args...)
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_using(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :using args
expr = Expr(:using, args...)
eval_ast(interpstate, mod, expr)
end
function interpret_ast_import(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :import args
expr = Expr(:import, args...)
eval_ast(interpstate, mod, expr)
end
function interpret_ast_export(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :export args
expr = Expr(:export, args...)
# Can we really lower exports?
eval_lower_ast(interpstate, mod, expr)
end
function interpret_ast_error(interpstate::InterpState, mod, args)
@show mod :error args
end
function interpret_ast_incomplete(interpstate, mod, args)
@show mod :incomplete args
end
function interpret_ast_toplevel(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :toplevel args
local linenumbernode
try
local ans
for arg in args
ans = interpret_ast_node(interpstate, mod, arg)
if ans isa LineNumberNode
linenumbernode = ans
end
end
ans
catch
@show linenumbernode
rethrow()
end
end
function interpret_ast_module(interpstate::InterpState, mod, args)
interpstate.options.debug && @show mod :module args
expr = Expr(:module, args...)
eval_ast(interpstate, mod, expr)
end
function interpret_ast_expr(interpstate::InterpState, mod, expr::Expr)
if expr.head == :comparison
interpret_ast_comparison(interpstate, mod, expr.args)
elseif expr.head == :(||)
interpret_ast_or(interpstate, mod, expr.args)
elseif expr.head == :(&&)
interpret_ast_and(interpstate, mod, expr.args)
elseif expr.head == :primitive
interpret_ast_primitive(interpstate, mod, expr.args)
elseif expr.head == :call
interpret_ast_call(interpstate, mod, expr.args)
elseif expr.head == :macrocall
interpret_ast_macrocall(interpstate, mod, expr.args)
elseif expr.head == :(.=)
interpret_ast_broadcast(interpstate, mod, expr.args)
elseif expr.head == :(=)
interpret_ast_assign(interpstate, mod, expr.args)
elseif expr.head == :const
interpret_ast_const(interpstate, mod, expr.args)
elseif expr.head == :local
interpret_ast_local(interpstate, mod, expr.args)
elseif expr.head == :global
interpret_ast_global(interpstate, mod, expr.args)
elseif expr.head == :let
interpret_ast_let(interpstate, mod, expr.args)
elseif expr.head == :block
interpret_ast_block(interpstate, mod, expr.args)
elseif expr.head == :try
interpret_ast_try(interpstate, mod, expr.args)
elseif expr.head == :do
interpret_ast_do(interpstate, mod, expr.args)
elseif expr.head == :if
interpret_ast_if(interpstate, mod, expr.args)
elseif expr.head == :for
interpret_ast_for(interpstate, mod, expr.args)
elseif expr.head == :while
interpret_ast_while(interpstate, mod, expr.args)
elseif expr.head == :function
interpret_ast_function(interpstate, mod, expr.args)
elseif expr.head == :where
interpret_ast_where(interpstate, mod, expr.args)
elseif expr.head == :macro
interpret_ast_macro(interpstate, mod, expr.args)
elseif expr.head == :struct
interpret_ast_struct(interpstate, mod, expr.args)
elseif expr.head == :curly
interpret_ast_curly(interpstate, mod, expr.args)
elseif expr.head == :abstract
interpret_ast_abstract(interpstate, mod, expr.args)
elseif expr.head == :using
interpret_ast_using(interpstate, mod, expr.args)
elseif expr.head == :import
interpret_ast_import(interpstate, mod, expr.args)
elseif expr.head == :export
interpret_ast_export(interpstate, mod, expr.args)
elseif expr.head == :error
interpret_ast_error(interpstate, mod, expr.args)
elseif expr.head == :incomplete
interpret_ast_incomplete(interpstate, mod, expr.args)
elseif expr.head == :toplevel
interpret_ast_toplevel(interpstate, mod, expr.args)
elseif expr.head == :module
interpret_ast_module(interpstate, mod, expr.args)
else
@show expr
@assert false
end
end
function interpret_ast_node(interpstate::InterpState, mod, node)
@nospecialize node
if node isa Expr
expr = node
interpret_ast_expr(interpstate, mod, expr)
elseif node isa Symbol
symbol = node
eval_ast(interpstate, mod, symbol)
elseif node isa LineNumberNode
linenumbernode = node
linenumbernode
else
@show typeof(node) node
@assert false
end
end
function interpret_ast(mod, expr, options)
interpstate = interp_state(mod, options)
try
interpret_ast_node(interpstate, mod, expr)
finally
#= for (wt, (meth, sparam_vals, src)) in interpstate.meths
@show wt meth sparam_vals
end =#
if interpstate.options.stats
for (mod_or_meth, stats) in sort(collect(interpstate.stats), by=kv -> kv[2].elapsed)
@show mod_or_meth Int(stats.calls) Int(stats.elapsed)
end
end
end
end
| [
8818,
5418,
62,
459,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
44052,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
1058,
18206,
62,
459,
953,
44052,
198,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
9093,
796,
7231,
13,
18206,
7,
4666,
11,
44052,
8,
198,
220,
220,
220,
220,
220,
220,
220,
9093,
796,
2488,
18206,
953,
720,
31937,
198,
220,
220,
220,
220,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
1058,
18206,
62,
459,
9093,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
9093,
198,
220,
220,
220,
4929,
6631,
198,
220,
220,
220,
220,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
1058,
18206,
62,
459,
953,
44052,
6631,
198,
220,
220,
220,
220,
220,
220,
220,
302,
16939,
3419,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
44052,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
1058,
18206,
62,
21037,
62,
459,
953,
44052,
198,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
300,
18351,
796,
30277,
13,
21037,
7,
4666,
11,
44052,
2599,
25,
3109,
1050,
198,
220,
220,
220,
220,
220,
220,
220,
611,
300,
18351,
13,
2256,
6624,
1058,
400,
2954,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
14873,
44146,
796,
2438,
62,
5219,
62,
6738,
62,
400,
2954,
7,
3849,
79,
5219,
11,
953,
11,
300,
18351,
13,
22046,
58,
16,
60,
3712,
14055,
13,
10669,
12360,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
9093,
796,
6179,
62,
21037,
7,
19815,
44146,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
12860,
300,
18351,
953,
44052,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1303,
9093,
796,
7231,
13,
18206,
7,
4666,
11,
44052,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
9093,
796,
2488,
18206,
953,
720,
31937,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
1058,
18206,
62,
21037,
62,
459,
9093,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
9093,
198,
220,
220,
220,
4929,
6631,
198,
220,
220,
220,
220,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
1058,
18206,
62,
21037,
62,
459,
953,
44052,
6631,
198,
220,
220,
220,
220,
220,
220,
220,
302,
16939,
3419,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
6179,
62,
459,
62,
785,
1845,
1653,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
785,
1845,
1653,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
785,
1845,
1653,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
198,
8818,
6179,
62,
459,
62,
273,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
36147,
15886,
8,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
37498,
15886,
828,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
392,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
36147,
25226,
8,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
37498,
25226,
828,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
198,
8818,
6179,
62,
459,
62,
19795,
1800,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
19795,
1800,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
19795,
1800,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
13345,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
13345,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
13345,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
20285,
12204,
439,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
20285,
12204,
439,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
20285,
12204,
439,
11,
26498,
23029,
198,
220,
220,
220,
1303,
4100,
4951,
307,
1365,
12118,
416,
262,
17050,
30,
198,
220,
220,
220,
5418,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
36654,
2701,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
36147,
13,
28,
8,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
37498,
13,
28,
828,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
562,
570,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
36147,
28,
8,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
37498,
28,
828,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
9979,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
9979,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
9979,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
12001,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
12001,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
12001,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
20541,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
20541,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
20541,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
1616,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
1616,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
1616,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
9967,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
9967,
26498,
198,
220,
220,
220,
1957,
41822,
4494,
17440,
198,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
1957,
9093,
198,
220,
220,
220,
220,
220,
220,
220,
329,
1822,
287,
26498,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
9093,
796,
6179,
62,
459,
62,
17440,
7,
3849,
79,
5219,
11,
953,
11,
1822,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
9093,
318,
64,
6910,
15057,
19667,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
41822,
4494,
17440,
796,
9093,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
9093,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
4929,
220,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
12860,
41822,
4494,
17440,
198,
220,
220,
220,
220,
220,
220,
220,
302,
16939,
3419,
198,
220,
220,
220,
886,
198,
437,
198,
8818,
6179,
62,
459,
62,
28311,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
28311,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
28311,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
4598,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
4598,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
4598,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
361,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
361,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
361,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
1640,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
1640,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
1640,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
4514,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
4514,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
4514,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
8818,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
8818,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
8818,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
3003,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
8818,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
3003,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
20285,
305,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
20285,
305,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
20285,
305,
11,
26498,
23029,
198,
220,
220,
220,
1303,
4100,
4951,
307,
1365,
12118,
416,
262,
17050,
30,
198,
220,
220,
220,
5418,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
7249,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
7249,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
7249,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
22019,
306,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
22019,
306,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
22019,
306,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
397,
8709,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
397,
8709,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
397,
8709,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
3500,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
3500,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
3500,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
11748,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
11748,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
11748,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
8818,
6179,
62,
459,
62,
39344,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
39344,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
39344,
11,
26498,
23029,
198,
220,
220,
220,
1303,
1680,
356,
1107,
2793,
15319,
30,
198,
220,
220,
220,
5418,
62,
21037,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
198,
8818,
6179,
62,
459,
62,
18224,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
2488,
12860,
953,
1058,
18224,
26498,
198,
437,
198,
8818,
6179,
62,
459,
62,
259,
20751,
7,
3849,
79,
5219,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
2488,
12860,
953,
1058,
259,
20751,
26498,
198,
437,
198,
8818,
6179,
62,
459,
62,
83,
643,
626,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
83,
643,
626,
26498,
198,
220,
220,
220,
1957,
41822,
4494,
17440,
198,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
1957,
9093,
198,
220,
220,
220,
220,
220,
220,
220,
329,
1822,
287,
26498,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
9093,
796,
6179,
62,
459,
62,
17440,
7,
3849,
79,
5219,
11,
953,
11,
1822,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
611,
9093,
318,
64,
6910,
15057,
19667,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
41822,
4494,
17440,
796,
9093,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
220,
220,
220,
220,
9093,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
198,
220,
220,
220,
4929,
220,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
12860,
41822,
4494,
17440,
198,
220,
220,
220,
220,
220,
220,
220,
302,
16939,
3419,
198,
220,
220,
220,
886,
198,
437,
198,
8818,
6179,
62,
459,
62,
21412,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
26498,
8,
198,
220,
220,
220,
987,
79,
5219,
13,
25811,
13,
24442,
11405,
2488,
12860,
953,
1058,
21412,
26498,
198,
220,
220,
220,
44052,
796,
1475,
1050,
7,
25,
21412,
11,
26498,
23029,
198,
220,
220,
220,
5418,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
437,
198,
198,
8818,
6179,
62,
459,
62,
31937,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
44052,
3712,
3109,
1050,
8,
198,
220,
220,
220,
611,
44052,
13,
2256,
6624,
1058,
785,
1845,
1653,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
785,
1845,
1653,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
36147,
15886,
8,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
273,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
36147,
25226,
8,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
392,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
19795,
1800,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
19795,
1800,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
13345,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
13345,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
20285,
12204,
439,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
20285,
12204,
439,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
36147,
13,
28,
8,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
36654,
2701,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
36147,
28,
8,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
562,
570,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
9979,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
9979,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
12001,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
12001,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
20541,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
20541,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
1616,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
1616,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
9967,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
9967,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
28311,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
28311,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
4598,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
4598,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
361,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
361,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
1640,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
1640,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
4514,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
4514,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
8818,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
8818,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
3003,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
3003,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
20285,
305,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
20285,
305,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
7249,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
7249,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
22019,
306,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
22019,
306,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
397,
8709,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
397,
8709,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
3500,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
3500,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
11748,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
11748,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
39344,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
39344,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
18224,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
18224,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
259,
20751,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
259,
20751,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
83,
643,
626,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
83,
643,
626,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
361,
44052,
13,
2256,
6624,
1058,
21412,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
21412,
7,
3849,
79,
5219,
11,
953,
11,
44052,
13,
22046,
8,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
12860,
44052,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
30493,
3991,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
6179,
62,
459,
62,
17440,
7,
3849,
79,
5219,
3712,
9492,
79,
9012,
11,
953,
11,
10139,
8,
198,
220,
220,
220,
2488,
39369,
431,
2413,
1096,
10139,
198,
220,
220,
220,
611,
10139,
318,
64,
1475,
1050,
198,
220,
220,
220,
220,
220,
220,
220,
44052,
796,
10139,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
31937,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
220,
220,
220,
2073,
361,
10139,
318,
64,
38357,
198,
220,
220,
220,
220,
220,
220,
220,
6194,
796,
10139,
198,
220,
220,
220,
220,
220,
220,
220,
5418,
62,
459,
7,
3849,
79,
5219,
11,
953,
11,
6194,
8,
198,
220,
220,
220,
2073,
361,
10139,
318,
64,
6910,
15057,
19667,
198,
220,
220,
220,
220,
220,
220,
220,
41822,
4494,
17440,
796,
10139,
198,
220,
220,
220,
220,
220,
220,
220,
41822,
4494,
17440,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
12860,
2099,
1659,
7,
17440,
8,
10139,
198,
220,
220,
220,
220,
220,
220,
220,
2488,
30493,
3991,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
6179,
62,
459,
7,
4666,
11,
44052,
11,
3689,
8,
198,
220,
220,
220,
987,
79,
5219,
796,
987,
79,
62,
5219,
7,
4666,
11,
3689,
8,
198,
220,
220,
220,
1949,
198,
220,
220,
220,
220,
220,
220,
220,
6179,
62,
459,
62,
17440,
7,
3849,
79,
5219,
11,
953,
11,
44052,
8,
198,
220,
220,
220,
3443,
198,
220,
220,
220,
220,
220,
220,
220,
1303,
28,
329,
357,
46569,
11,
357,
76,
2788,
11,
37331,
321,
62,
12786,
11,
12351,
4008,
287,
987,
79,
5219,
13,
76,
2788,
82,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
12860,
266,
83,
11248,
37331,
321,
62,
12786,
198,
220,
220,
220,
220,
220,
220,
220,
886,
796,
2,
198,
220,
220,
220,
220,
220,
220,
220,
611,
987,
79,
5219,
13,
25811,
13,
34242,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
329,
357,
4666,
62,
273,
62,
76,
2788,
11,
9756,
8,
287,
3297,
7,
33327,
7,
3849,
79,
5219,
13,
34242,
828,
416,
28,
74,
85,
4613,
479,
85,
58,
17,
4083,
417,
28361,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2488,
12860,
953,
62,
273,
62,
76,
2788,
2558,
7,
34242,
13,
66,
5691,
8,
2558,
7,
34242,
13,
417,
28361,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
886,
220,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
437,
198
] | 2.49515 | 4,639 |
module Apply
export apply, offset_apply
using Base: tail
apply(f::F, a::Tuple{A,Vararg}, args...) where {F,A} =
(f(a[1], args...), apply(f, tail(a), args...)...)
apply(f::F, a::Tuple{A,Vararg}, b::Tuple{B,Vararg}, args...) where {F,A,B} =
(f(a[1], b[1], args...), apply(f, tail(a), tail(b), args...)...)
apply(f::F, a::Tuple{A,Vararg}, b::Tuple{B,Vararg}, c::Tuple{C,Vararg}, args...) where {F,A,B,C} =
(f(a[1], b[1], c[1], args...), apply(f, tail(a), tail(b), tail(c), args...)...)
apply(f, ::Tuple{}, ::Tuple{}, ::Tuple{}, args...) = ()
apply(f, a, ::Tuple{}, ::Tuple{}, args...) = ()
apply(f, ::Tuple{}, b, ::Tuple{}, args...) = ()
apply(f, a, b, ::Tuple{}, args...) = ()
apply(f, ::Tuple{}, ::Tuple{}, args...) = ()
apply(f, a, ::Tuple{}, args...) = ()
apply(f, ::Tuple{}, args...) = ()
offset_apply(f, o::Tuple{O,Vararg}, a::AbstractArray, offset::Int, args...) where O = begin
offset = f(o[1], a, offset, args...)
offset_apply(f, tail(o), a, offset::Int, args...)
nothing
end
offset_apply(f, a::AbstractArray, o::Tuple{O,Vararg}, offset::Int, args...) where O = begin
offset = f(a, o[1], offset, args...)
offset_apply(f, a, tail(o), offset::Int, args...)
nothing
end
offset_apply(f, o::Tuple{O,Vararg}, offset::Int, args...) where O = begin
offset = f(o[1], offset, args...)
offset_apply(f, tail(o), offset::Int, args...)
nothing
end
offset_apply(f, o::Tuple{}, offset::Int, args...) = nothing
offset_apply(f, a::AbstractArray, o::Tuple{}, offset::Int, args...) = nothing
offset_apply(f, o::Tuple{}, a::AbstractArray, offset::Int, args...) = nothing
end # module
| [
21412,
27967,
198,
198,
39344,
4174,
11,
11677,
62,
39014,
198,
3500,
7308,
25,
7894,
198,
198,
39014,
7,
69,
3712,
37,
11,
257,
3712,
51,
29291,
90,
32,
11,
19852,
853,
5512,
26498,
23029,
810,
1391,
37,
11,
32,
92,
796,
220,
198,
220,
220,
220,
357,
69,
7,
64,
58,
16,
4357,
26498,
986,
828,
4174,
7,
69,
11,
7894,
7,
64,
828,
26498,
23029,
23029,
198,
39014,
7,
69,
3712,
37,
11,
257,
3712,
51,
29291,
90,
32,
11,
19852,
853,
5512,
275,
3712,
51,
29291,
90,
33,
11,
19852,
853,
5512,
26498,
23029,
810,
1391,
37,
11,
32,
11,
33,
92,
796,
220,
198,
220,
220,
220,
357,
69,
7,
64,
58,
16,
4357,
275,
58,
16,
4357,
26498,
986,
828,
4174,
7,
69,
11,
7894,
7,
64,
828,
7894,
7,
65,
828,
26498,
23029,
23029,
198,
39014,
7,
69,
3712,
37,
11,
257,
3712,
51,
29291,
90,
32,
11,
19852,
853,
5512,
275,
3712,
51,
29291,
90,
33,
11,
19852,
853,
5512,
269,
3712,
51,
29291,
90,
34,
11,
19852,
853,
5512,
26498,
23029,
810,
1391,
37,
11,
32,
11,
33,
11,
34,
92,
796,
220,
198,
220,
220,
220,
357,
69,
7,
64,
58,
16,
4357,
275,
58,
16,
4357,
269,
58,
16,
4357,
26498,
986,
828,
4174,
7,
69,
11,
7894,
7,
64,
828,
7894,
7,
65,
828,
7894,
7,
66,
828,
26498,
23029,
23029,
198,
198,
39014,
7,
69,
11,
7904,
51,
29291,
90,
5512,
7904,
51,
29291,
90,
5512,
7904,
51,
29291,
90,
5512,
26498,
23029,
796,
7499,
198,
39014,
7,
69,
11,
257,
11,
7904,
51,
29291,
90,
5512,
7904,
51,
29291,
90,
5512,
26498,
23029,
796,
7499,
198,
39014,
7,
69,
11,
7904,
51,
29291,
90,
5512,
275,
11,
7904,
51,
29291,
90,
5512,
26498,
23029,
796,
7499,
198,
39014,
7,
69,
11,
257,
11,
275,
11,
7904,
51,
29291,
90,
5512,
26498,
23029,
796,
7499,
198,
39014,
7,
69,
11,
7904,
51,
29291,
90,
5512,
7904,
51,
29291,
90,
5512,
26498,
23029,
796,
7499,
198,
39014,
7,
69,
11,
257,
11,
7904,
51,
29291,
90,
5512,
26498,
23029,
796,
7499,
198,
39014,
7,
69,
11,
7904,
51,
29291,
90,
5512,
26498,
23029,
796,
7499,
628,
198,
28968,
62,
39014,
7,
69,
11,
267,
3712,
51,
29291,
90,
46,
11,
19852,
853,
5512,
257,
3712,
23839,
19182,
11,
11677,
3712,
5317,
11,
26498,
23029,
810,
440,
796,
2221,
198,
220,
220,
220,
11677,
796,
277,
7,
78,
58,
16,
4357,
257,
11,
11677,
11,
26498,
23029,
198,
220,
220,
220,
11677,
62,
39014,
7,
69,
11,
7894,
7,
78,
828,
257,
11,
11677,
3712,
5317,
11,
26498,
23029,
198,
220,
220,
220,
2147,
198,
437,
198,
28968,
62,
39014,
7,
69,
11,
257,
3712,
23839,
19182,
11,
267,
3712,
51,
29291,
90,
46,
11,
19852,
853,
5512,
11677,
3712,
5317,
11,
26498,
23029,
810,
440,
796,
2221,
198,
220,
220,
220,
11677,
796,
277,
7,
64,
11,
267,
58,
16,
4357,
11677,
11,
26498,
23029,
198,
220,
220,
220,
11677,
62,
39014,
7,
69,
11,
257,
11,
7894,
7,
78,
828,
11677,
3712,
5317,
11,
26498,
23029,
198,
220,
220,
220,
2147,
198,
437,
198,
28968,
62,
39014,
7,
69,
11,
267,
3712,
51,
29291,
90,
46,
11,
19852,
853,
5512,
11677,
3712,
5317,
11,
26498,
23029,
810,
440,
796,
2221,
198,
220,
220,
220,
11677,
796,
277,
7,
78,
58,
16,
4357,
11677,
11,
26498,
23029,
198,
220,
220,
220,
11677,
62,
39014,
7,
69,
11,
7894,
7,
78,
828,
11677,
3712,
5317,
11,
26498,
23029,
198,
220,
220,
220,
2147,
198,
437,
198,
28968,
62,
39014,
7,
69,
11,
267,
3712,
51,
29291,
90,
5512,
11677,
3712,
5317,
11,
26498,
23029,
796,
2147,
198,
28968,
62,
39014,
7,
69,
11,
257,
3712,
23839,
19182,
11,
267,
3712,
51,
29291,
90,
5512,
11677,
3712,
5317,
11,
26498,
23029,
796,
2147,
198,
28968,
62,
39014,
7,
69,
11,
267,
3712,
51,
29291,
90,
5512,
257,
3712,
23839,
19182,
11,
11677,
3712,
5317,
11,
26498,
23029,
796,
2147,
198,
198,
437,
1303,
8265,
198
] | 2.412463 | 674 |
using Documenter, ICD10Utilities
makedocs(
sitename = "ICD-10 Utilities",
modules=[ICD10Utilities],
pages=[
"ICD-10" => "index.md",
"icdo3.md",
"icd10am.md",
"icd10cm.md"
])
deploydocs(
repo = "github.com/timbp/ICD10Utilities.jl.git",
devbranch = "main",
)
| [
3500,
16854,
263,
11,
314,
8610,
940,
18274,
2410,
198,
198,
76,
4335,
420,
82,
7,
198,
197,
1650,
12453,
796,
366,
2149,
35,
12,
940,
41086,
1600,
198,
197,
13103,
41888,
2149,
35,
940,
18274,
2410,
4357,
198,
220,
220,
5468,
41888,
198,
220,
220,
220,
220,
366,
2149,
35,
12,
940,
1,
5218,
366,
9630,
13,
9132,
1600,
198,
220,
220,
220,
220,
366,
291,
4598,
18,
13,
9132,
1600,
198,
220,
220,
220,
220,
366,
291,
67,
940,
321,
13,
9132,
1600,
198,
220,
220,
220,
220,
366,
291,
67,
940,
11215,
13,
9132,
1,
198,
220,
220,
33761,
198,
198,
2934,
1420,
31628,
7,
198,
220,
220,
220,
29924,
796,
366,
12567,
13,
785,
14,
83,
14107,
79,
14,
2149,
35,
940,
18274,
2410,
13,
20362,
13,
18300,
1600,
198,
220,
220,
220,
1614,
1671,
3702,
796,
366,
12417,
1600,
198,
8,
198
] | 2.020408 | 147 |
function ShortPeriodicsInterpolatedCoefficient(arg0::jint)
return ShortPeriodicsInterpolatedCoefficient((jint,), arg0)
end
function add_grid_point(obj::ShortPeriodicsInterpolatedCoefficient, arg0::AbsoluteDate, arg1::Vector{jdouble})
return jcall(obj, "addGridPoint", void, (AbsoluteDate, Vector{jdouble}), arg0, arg1)
end
function clear_history(obj::ShortPeriodicsInterpolatedCoefficient)
return jcall(obj, "clearHistory", void, ())
end
function value(obj::ShortPeriodicsInterpolatedCoefficient, arg0::AbsoluteDate)
return jcall(obj, "value", Vector{jdouble}, (AbsoluteDate,), arg0)
end
| [
8818,
10073,
5990,
2101,
873,
9492,
16104,
515,
34,
2577,
5632,
7,
853,
15,
3712,
73,
600,
8,
198,
220,
220,
220,
1441,
10073,
5990,
2101,
873,
9492,
16104,
515,
34,
2577,
5632,
19510,
73,
600,
11,
828,
1822,
15,
8,
198,
437,
198,
198,
8818,
751,
62,
25928,
62,
4122,
7,
26801,
3712,
16438,
5990,
2101,
873,
9492,
16104,
515,
34,
2577,
5632,
11,
1822,
15,
3712,
24849,
3552,
10430,
11,
1822,
16,
3712,
38469,
90,
73,
23352,
30072,
198,
220,
220,
220,
1441,
474,
13345,
7,
26801,
11,
366,
2860,
41339,
12727,
1600,
7951,
11,
357,
24849,
3552,
10430,
11,
20650,
90,
73,
23352,
92,
828,
1822,
15,
11,
1822,
16,
8,
198,
437,
198,
198,
8818,
1598,
62,
23569,
7,
26801,
3712,
16438,
5990,
2101,
873,
9492,
16104,
515,
34,
2577,
5632,
8,
198,
220,
220,
220,
1441,
474,
13345,
7,
26801,
11,
366,
20063,
18122,
1600,
7951,
11,
32865,
198,
437,
198,
198,
8818,
1988,
7,
26801,
3712,
16438,
5990,
2101,
873,
9492,
16104,
515,
34,
2577,
5632,
11,
1822,
15,
3712,
24849,
3552,
10430,
8,
198,
220,
220,
220,
1441,
474,
13345,
7,
26801,
11,
366,
8367,
1600,
20650,
90,
73,
23352,
5512,
357,
24849,
3552,
10430,
11,
828,
1822,
15,
8,
198,
437,
628
] | 2.895238 | 210 |
function cartesianmoveindex(direction)
directiondict = Dict("UP" => (-1, 0), "DOWN" => (1, 0), "LEFT" => (0, -1), "RIGHT" => (0, 1))
return CartesianIndex(directiondict[direction])
end
function swaptiles!(board, x, y)
temp = board[x]
board[x] = board[y]
board[y] = temp
end
function moves(board)
first = findfirst(iszero, board)
movedict = Dict("UP" => "DOWN", "DOWN" => "UP", "LEFT" => "RIGHT", "RIGHT" => "LEFT")
directions = []
for i in ("UP", "DOWN", "LEFT", "RIGHT")
index = first + cartesianmoveindex(i)
if checkbounds(Bool, board, index)
action = (index, movedict[i])
push!(directions, action)
end
end
return directions
end
function copyboard(action, board)
newboard = copy(board)
index1 = action[1]
index2 = index1 + cartesianmoveindex(action[2])
swaptiles!(newboard, index1, index2)
return newboard
end
## determine all options for move states
function expansion(board)
states = []
possacts = moves(board)
for act in possacts
push!(states, copyboard(act, board))
end
return states
end
| [
8818,
6383,
35610,
21084,
9630,
7,
37295,
8,
198,
220,
220,
220,
4571,
11600,
796,
360,
713,
7203,
8577,
1,
5218,
13841,
16,
11,
657,
828,
366,
41925,
1,
5218,
357,
16,
11,
657,
828,
366,
2538,
9792,
1,
5218,
357,
15,
11,
532,
16,
828,
366,
49,
9947,
1,
5218,
357,
15,
11,
352,
4008,
628,
220,
220,
220,
1441,
13690,
35610,
15732,
7,
37295,
11600,
58,
37295,
12962,
198,
437,
628,
198,
8818,
1509,
2373,
2915,
0,
7,
3526,
11,
2124,
11,
331,
8,
198,
220,
220,
220,
20218,
796,
3096,
58,
87,
60,
198,
220,
220,
220,
3096,
58,
87,
60,
796,
3096,
58,
88,
60,
198,
220,
220,
220,
3096,
58,
88,
60,
796,
20218,
198,
437,
628,
198,
8818,
6100,
7,
3526,
8,
198,
220,
220,
220,
717,
796,
1064,
11085,
7,
271,
22570,
11,
3096,
8,
198,
220,
220,
220,
3888,
713,
796,
360,
713,
7203,
8577,
1,
5218,
366,
41925,
1600,
366,
41925,
1,
5218,
366,
8577,
1600,
366,
2538,
9792,
1,
5218,
366,
49,
9947,
1600,
366,
49,
9947,
1,
5218,
366,
2538,
9792,
4943,
628,
220,
220,
220,
11678,
796,
17635,
198,
220,
220,
220,
329,
1312,
287,
5855,
8577,
1600,
366,
41925,
1600,
366,
2538,
9792,
1600,
366,
49,
9947,
4943,
198,
220,
220,
220,
220,
220,
220,
220,
6376,
796,
717,
1343,
6383,
35610,
21084,
9630,
7,
72,
8,
628,
220,
220,
220,
220,
220,
220,
220,
611,
2198,
65,
3733,
7,
33,
970,
11,
3096,
11,
6376,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
2223,
796,
357,
9630,
11,
3888,
713,
58,
72,
12962,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
12942,
507,
11,
2223,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1441,
11678,
198,
437,
628,
198,
8818,
4866,
3526,
7,
2673,
11,
3096,
8,
198,
220,
220,
220,
649,
3526,
796,
4866,
7,
3526,
8,
198,
220,
220,
220,
6376,
16,
796,
2223,
58,
16,
60,
198,
220,
220,
220,
6376,
17,
796,
6376,
16,
1343,
6383,
35610,
21084,
9630,
7,
2673,
58,
17,
12962,
198,
220,
220,
220,
1509,
2373,
2915,
0,
7,
3605,
3526,
11,
6376,
16,
11,
6376,
17,
8,
628,
220,
220,
220,
1441,
649,
3526,
198,
437,
198,
198,
2235,
5004,
477,
3689,
329,
1445,
2585,
198,
8818,
7118,
7,
3526,
8,
198,
220,
220,
220,
2585,
796,
17635,
198,
220,
220,
220,
1184,
8656,
796,
6100,
7,
3526,
8,
628,
220,
220,
220,
329,
719,
287,
1184,
8656,
198,
220,
220,
220,
220,
220,
220,
220,
4574,
0,
7,
27219,
11,
4866,
3526,
7,
529,
11,
3096,
4008,
198,
220,
220,
220,
886,
628,
220,
220,
220,
1441,
2585,
198,
437,
198
] | 2.452991 | 468 |
######################################################################
# weierstrasspoints.jl: Addition laws for projective points on Weierstrass curves
######################################################################
export infinity, projective_add, projective_scalar_mul
######################################################################
# Basic methods
######################################################################
"""
Get the point at infinity on an elliptic curve in Weierstrass form.
"""
function infinity(E::AbstractWeierstrass)
R = base_ring(E)
return EllipticPoint(Nemo.zero(R), Nemo.one(R), Nemo.zero(R), E)
end
function zero(E::AbstractWeierstrass)
return infinity(E)
end
######################################################################
# Addition law
######################################################################
"""
Get the opposite of a point on an elliptic curve in Weierstrass form.
"""
function -(P::EllipticPoint)
E = P.curve
x, y, z = coordinates(P)
a1, _, a3, _, _ = a_invariants(E)
return EllipticPoint(x, - y - a1 * x - a3 * z, z, E)
end
"""
Get the sum of two normalized projective points on the same Weierstrass curve, assuming they are not equal and not inverse of each other.
"""
function _addgeneric(P::EllipticPoint{T}, Q::EllipticPoint{T}) where T<:Nemo.FieldElem
E = P.curve
a1, a2, a3, _, _ = a_invariants(E)
xq, yq, zq = coordinates(Q)
@assert zq == 1
xp, yp, zp = coordinates(P)
@assert zp == 1
#sanity check
@assert xp != xq
denom = xq - xp
inverse = 1//denom
lambda = (yq - yp) * inverse
nu = (yp * xq - xp * yq) * inverse
Xplus = lambda^2 + a1 * lambda - a2 - xp - xq
Yplus = -(lambda + a1) * Xplus - nu - a3
Zplus = Nemo.one(base_ring(P))
return EllipticPoint(Xplus, Yplus, Zplus, E)
end
"""
Get the sum of two normalized projective points on the same Weierstrass curve, assuming they have equal x-coordinate.
"""
function _addequalx(P::EllipticPoint{T}, Q::EllipticPoint{T}) where T<:Nemo.FieldElem
E = P.curve
a1, a2, a3, a4, a6 = a_invariants(E)
xp, yp, zp = coordinates(P)
@assert zp == 1
xq, yq, zq = coordinates(Q)
@assert zq == 1
#sanity check
@assert xp == xq
denom = yp + yq + a1 * xq + a3
if iszero(denom)
return infinity(E)
else
inverse = 1//denom
lambda = (3 * xp^2 + 2 * a2 * xp + a4 - a1 * yp) * inverse
nu = (- xp^3 + a4 * xp + 2 * a6 - a3 * yp) * inverse
Xplus = lambda^2 + a1 * lambda - a2 - xp - xq
Yplus = -(lambda + a1) * Xplus - nu - a3
Zplus = one(base_ring(P))
return EllipticPoint(Xplus, Yplus, Zplus, E)
end
end
"""
Get the double of a normalized point.
"""
function _double(P::EllipticPoint)
if isinfinity(P)
return infinity(P.curve)
else
return _addequalx(P, P)
end
end
"""
Get the sum of two projective points on the same Weierstrass curve.
"""
function +(P::EllipticPoint{T}, Q::EllipticPoint{T}) where T<:Nemo.FieldElem
P = normalized(P)
Q = normalized(Q)
xp, _, _ = coordinates(P)
xq, _, _ = coordinates(Q)
if isinfinity(P)
return Q
elseif isinfinity(Q)
return P
elseif xp == xq
return _addequalx(P,Q)
else
return _addgeneric(P,Q)
end
end
function -(P::EllipticPoint{T}, Q::EllipticPoint{T}) where T<:FieldElem
return P + (-Q)
end
"""
Get a scalar multiple of a point on a Weierstrass curve.
"""
function *(k::Integer, P::EllipticPoint)
E = P.curve
P = normalized(P)
if k == 0
return infinity(E)
elseif k<0
return (-k) * (-P)
else
if isinfinity(P)
return infinity(E)
else
return _ladder(k, P)
end
end
end
# function *(k::Integer, P::EllipticPoint)
# return k * P
# end
function _ladder(m::Integer, P::EllipticPoint)
p0 = P
for b in Iterators.drop(Iterators.reverse(digits(Int8, m, base=2)), 1)
if (b == 0)
p0 = _double(p0)
else
p0 = P + _double(p0)
end
end
return p0
end
######################################################################
# Projective addition law for Short Weierstrass curves
######################################################################
# P = (x1, y1, z1)
# Q = (x2, y2, z2)
# P + Q = (x3, y3, z3)
# if P != Q then
# x3 = (x2 z1 - x1 z2) [ (y2 z1 - y1 z2)^2 z1 z2 - (x2 z1 - x1 z2)^2 (x2 z1 + x1 z2) ]
# y3 = (y2 z1 - y1 z2) [ (x2 z1 - x1 z2)^2 (x2 z1 + 2 x1 z2) - (y2 z1 - y1 z2)^2 z1 z2 ] - (x2 z1 - x1 z2)^3 y1 z2
# z3 = (x2 z1 - x1 z2)^3 z1 z2
# if P = Q then
# x3 = 2 y1 z1 [ (a z1^2 + 3 x1^2)^2 - 8 x1 y1^2 z1 ]
# y3 = (a z1^2 + 3 x1^2 ) [ 12 x1 y1^2 z1 - a^2 (z1^2 + 3 x1^2)^2 ] - 8 y1^4 z1^2
# z3 = (2 y1 z1)^3
#This function is only to be used with distinct points on the same short Weierstrass curve
#xdet is x2 z1 - x1 z2, and ydet is y2 z1 - y1 z2
function _projective_add_neq(P::EllipticPoint{T}, Q::EllipticPoint{T}, xdet::T, ydet::T) where T
x1, y1, z1 = coordinates(P)
x2, y2, z2 = coordinates(Q)
xdet2 = xdet^2
xdet3 = xdet2 * xdet
ydet2 = ydet^2
z1z2 = z1 * z2
#we could save a few multiplications in what follows
x3 = xdet * ( ydet2 * z1z2 - xdet2 * (x2 * z1 + x1 * z2) )
y3 = ydet * ( xdet2 * (x2 * z1 + 2 * x1 * z2) - ydet2 * z1z2 ) - xdet3 * y1 * z2
z3 = xdet3 * z1z2
res = Point(x3, y3, z3, base_curve(P))
#@assert isvalid(res) #sanity check
return res
end
#This function is only to be used with a point on a short Weierstrass curve
function _projective_dbl(P::EllipticPoint{T}) where T
x, y, z = coordinates(P)
_, _, _, a, b = a_invariants(base_curve(P))
factor = a * z^2 + 3 * x^2
factor2 = factor^2
yz = y * z
xy2z = x * y * yz
#we could save again a few multiplications in what follows
xprime = 2 * yz * ( factor2 - 8 * xy2z )
yprime = factor * ( 12 * xy2z - factor2 ) - 8 * (yz)^2 * y^2
zprime = 8 * (yz)^3
res = Point(xprime, yprime, zprime, base_curve(P))
#@assert isvalid(res) #sanity check
return res
end
#This function is only to be used with two points on the same short Weierstrass curve
#Compute xdet and ydet, if they are both zero go to _projective_dbl
function projective_add(P::EllipticPoint{T}, Q::EllipticPoint{T}) where T
x1, y1, z1 = coordinates(P)
x2, y2, z2 = coordinates(Q)
xdet = x2 * z1 - x1 * z2
ydet = y2 * z1 - y1 * z2
if ((xdet == 0) & (ydet == 0))
return _projective_dbl(P)
else
return _projective_add_neq(P, Q, xdet, ydet)
end
end
#Here we assume v is a positive integer and P lives on a short Weierstrass curve
function _projective_scalar_mul(P::EllipticPoint, v::Integer)
P0 = P
for b in Iterators.drop(Iterators.reverse(digits(Int8, v, base=2)), 1)
P0 = _projective_dbl(P0)
if (b == 1)
P0 = projective_add(P0, P)
end
end
return P0
end
function projective_scalar_mul(P::EllipticPoint, v::Integer)
if v == 0
return infinity(base_curve(E))
elseif v < 0
return - _projective_scalar_mul(P, -v)
else
return _projective_scalar_mul(P, v)
end
end
| [
198,
29113,
29113,
4242,
2235,
198,
2,
356,
959,
2536,
562,
13033,
13,
20362,
25,
3060,
653,
3657,
329,
1628,
425,
2173,
319,
775,
959,
2536,
562,
23759,
198,
29113,
29113,
4242,
2235,
198,
198,
39344,
37174,
11,
1628,
425,
62,
2860,
11,
1628,
425,
62,
1416,
282,
283,
62,
76,
377,
628,
198,
29113,
29113,
4242,
2235,
198,
2,
14392,
5050,
198,
29113,
29113,
4242,
2235,
198,
198,
37811,
198,
3855,
262,
966,
379,
37174,
319,
281,
48804,
291,
12133,
287,
775,
959,
2536,
562,
1296,
13,
198,
37811,
198,
8818,
37174,
7,
36,
3712,
23839,
1135,
959,
2536,
562,
8,
198,
220,
220,
220,
371,
796,
2779,
62,
1806,
7,
36,
8,
198,
220,
220,
220,
1441,
7122,
10257,
291,
12727,
7,
45,
41903,
13,
22570,
7,
49,
828,
22547,
78,
13,
505,
7,
49,
828,
22547,
78,
13,
22570,
7,
49,
828,
412,
8,
198,
437,
198,
198,
8818,
6632,
7,
36,
3712,
23839,
1135,
959,
2536,
562,
8,
198,
197,
7783,
37174,
7,
36,
8,
198,
437,
628,
198,
29113,
29113,
4242,
2235,
198,
2,
3060,
653,
1099,
198,
29113,
29113,
4242,
2235,
198,
198,
37811,
198,
3855,
262,
6697,
286,
257,
966,
319,
281,
48804,
291,
12133,
287,
775,
959,
2536,
562,
1296,
13,
198,
37811,
198,
8818,
532,
7,
47,
3712,
30639,
10257,
291,
12727,
8,
198,
197,
36,
796,
350,
13,
22019,
303,
198,
197,
87,
11,
331,
11,
1976,
796,
22715,
7,
47,
8,
198,
197,
64,
16,
11,
4808,
11,
257,
18,
11,
4808,
11,
4808,
796,
257,
62,
16340,
2743,
1187,
7,
36,
8,
198,
220,
220,
220,
1441,
7122,
10257,
291,
12727,
7,
87,
11,
532,
331,
532,
257,
16,
1635,
2124,
532,
257,
18,
1635,
1976,
11,
1976,
11,
412,
8,
198,
437,
198,
198,
37811,
198,
3855,
262,
2160,
286,
734,
39279,
1628,
425,
2173,
319,
262,
976,
775,
959,
2536,
562,
12133,
11,
13148,
484,
389,
407,
4961,
290,
407,
34062,
286,
1123,
584,
13,
198,
37811,
198,
8818,
4808,
2860,
41357,
7,
47,
3712,
30639,
10257,
291,
12727,
90,
51,
5512,
1195,
3712,
30639,
10257,
291,
12727,
90,
51,
30072,
810,
309,
27,
25,
45,
41903,
13,
15878,
36,
10671,
198,
220,
220,
220,
412,
796,
350,
13,
22019,
303,
198,
197,
64,
16,
11,
257,
17,
11,
257,
18,
11,
4808,
11,
4808,
796,
257,
62,
16340,
2743,
1187,
7,
36,
8,
198,
197,
198,
197,
87,
80,
11,
331,
80,
11,
1976,
80,
796,
22715,
7,
48,
8,
198,
197,
31,
30493,
1976,
80,
6624,
352,
198,
197,
42372,
11,
331,
79,
11,
1976,
79,
796,
22715,
7,
47,
8,
198,
197,
31,
30493,
1976,
79,
6624,
352,
198,
197,
198,
197,
2,
12807,
414,
2198,
198,
197,
31,
30493,
36470,
14512,
2124,
80,
198,
197,
198,
220,
220,
220,
2853,
296,
796,
2124,
80,
532,
36470,
198,
197,
259,
4399,
796,
352,
1003,
6559,
296,
198,
220,
220,
220,
37456,
796,
357,
88,
80,
532,
331,
79,
8,
1635,
34062,
198,
220,
220,
220,
14364,
796,
357,
4464,
1635,
2124,
80,
532,
36470,
1635,
331,
80,
8,
1635,
34062,
198,
220,
220,
220,
220,
198,
220,
220,
220,
1395,
9541,
796,
37456,
61,
17,
1343,
257,
16,
1635,
37456,
532,
257,
17,
532,
36470,
532,
2124,
80,
198,
220,
220,
220,
575,
9541,
796,
532,
7,
50033,
1343,
257,
16,
8,
1635,
1395,
9541,
532,
14364,
532,
257,
18,
198,
220,
220,
220,
1168,
9541,
796,
22547,
78,
13,
505,
7,
8692,
62,
1806,
7,
47,
4008,
198,
220,
220,
220,
1441,
7122,
10257,
291,
12727,
7,
55,
9541,
11,
575,
9541,
11,
1168,
9541,
11,
412,
8,
198,
437,
198,
198,
37811,
198,
3855,
262,
2160,
286,
734,
39279,
1628,
425,
2173,
319,
262,
976,
775,
959,
2536,
562,
12133,
11,
13148,
484,
423,
4961,
2124,
12,
37652,
4559,
13,
198,
37811,
198,
8818,
4808,
2860,
40496,
87,
7,
47,
3712,
30639,
10257,
291,
12727,
90,
51,
5512,
1195,
3712,
30639,
10257,
291,
12727,
90,
51,
30072,
810,
309,
27,
25,
45,
41903,
13,
15878,
36,
10671,
198,
220,
220,
220,
412,
796,
350,
13,
22019,
303,
198,
197,
64,
16,
11,
257,
17,
11,
257,
18,
11,
257,
19,
11,
257,
21,
796,
257,
62,
16340,
2743,
1187,
7,
36,
8,
198,
197,
198,
197,
42372,
11,
331,
79,
11,
1976,
79,
796,
22715,
7,
47,
8,
198,
197,
31,
30493,
1976,
79,
6624,
352,
198,
197,
87,
80,
11,
331,
80,
11,
1976,
80,
796,
22715,
7,
48,
8,
198,
197,
31,
30493,
1976,
80,
6624,
352,
198,
197,
198,
197,
2,
12807,
414,
2198,
198,
197,
31,
30493,
36470,
6624,
2124,
80,
198,
197,
198,
220,
220,
220,
2853,
296,
796,
331,
79,
1343,
331,
80,
1343,
257,
16,
1635,
2124,
80,
1343,
257,
18,
198,
220,
220,
220,
611,
318,
22570,
7,
6559,
296,
8,
198,
197,
220,
220,
220,
1441,
37174,
7,
36,
8,
198,
220,
220,
220,
2073,
198,
197,
197,
259,
4399,
796,
352,
1003,
6559,
296,
198,
197,
220,
220,
220,
37456,
796,
357,
18,
1635,
36470,
61,
17,
1343,
362,
1635,
257,
17,
1635,
36470,
1343,
257,
19,
532,
257,
16,
1635,
331,
79,
8,
1635,
34062,
198,
197,
220,
220,
220,
14364,
796,
13841,
36470,
61,
18,
1343,
257,
19,
1635,
36470,
1343,
362,
1635,
257,
21,
532,
257,
18,
1635,
331,
79,
8,
1635,
34062,
198,
197,
220,
220,
220,
1395,
9541,
796,
37456,
61,
17,
1343,
257,
16,
1635,
37456,
532,
257,
17,
532,
36470,
532,
2124,
80,
198,
220,
220,
220,
220,
220,
220,
220,
575,
9541,
796,
532,
7,
50033,
1343,
257,
16,
8,
1635,
1395,
9541,
532,
14364,
532,
257,
18,
198,
220,
220,
220,
220,
220,
220,
220,
1168,
9541,
796,
530,
7,
8692,
62,
1806,
7,
47,
4008,
198,
197,
220,
220,
220,
1441,
7122,
10257,
291,
12727,
7,
55,
9541,
11,
575,
9541,
11,
1168,
9541,
11,
412,
8,
198,
220,
220,
220,
886,
198,
437,
198,
198,
37811,
198,
3855,
262,
4274,
286,
257,
39279,
966,
13,
198,
37811,
198,
8818,
4808,
23352,
7,
47,
3712,
30639,
10257,
291,
12727,
8,
198,
197,
361,
318,
10745,
6269,
7,
47,
8,
198,
197,
197,
7783,
37174,
7,
47,
13,
22019,
303,
8,
198,
197,
17772,
198,
197,
197,
7783,
4808,
2860,
40496,
87,
7,
47,
11,
350,
8,
198,
197,
437,
198,
437,
628,
198,
37811,
198,
3855,
262,
2160,
286,
734,
1628,
425,
2173,
319,
262,
976,
775,
959,
2536,
562,
12133,
13,
198,
198,
37811,
198,
8818,
1343,
7,
47,
3712,
30639,
10257,
291,
12727,
90,
51,
5512,
1195,
3712,
30639,
10257,
291,
12727,
90,
51,
30072,
810,
309,
27,
25,
45,
41903,
13,
15878,
36,
10671,
198,
220,
220,
220,
350,
796,
39279,
7,
47,
8,
198,
220,
220,
220,
1195,
796,
39279,
7,
48,
8,
198,
197,
42372,
11,
4808,
11,
4808,
796,
22715,
7,
47,
8,
198,
197,
87,
80,
11,
4808,
11,
4808,
796,
22715,
7,
48,
8,
198,
220,
220,
220,
611,
318,
10745,
6269,
7,
47,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
1195,
198,
220,
220,
220,
2073,
361,
318,
10745,
6269,
7,
48,
8,
198,
220,
220,
220,
220,
220,
220,
220,
1441,
350,
198,
220,
220,
220,
2073,
361,
36470,
6624,
2124,
80,
198,
197,
197,
7783,
4808,
2860,
40496,
87,
7,
47,
11,
48,
8,
198,
220,
220,
220,
2073,
198,
197,
197,
7783,
4808,
2860,
41357,
7,
47,
11,
48,
8,
198,
220,
220,
220,
886,
198,
437,
198,
198,
8818,
532,
7,
47,
3712,
30639,
10257,
291,
12727,
90,
51,
5512,
1195,
3712,
30639,
10257,
291,
12727,
90,
51,
30072,
810,
309,
27,
25,
15878,
36,
10671,
198,
197,
7783,
350,
1343,
13841,
48,
8,
198,
437,
198,
198,
37811,
198,
3855,
257,
16578,
283,
3294,
286,
257,
966,
319,
257,
775,
959,
2536,
562,
12133,
13,
198,
37811,
198,
198,
8818,
1635,
7,
74,
3712,
46541,
11,
350,
3712,
30639,
10257,
291,
12727,
8,
198,
197,
36,
796,
350,
13,
22019,
303,
198,
197,
47,
796,
39279,
7,
47,
8,
198,
197,
361,
479,
6624,
657,
198,
197,
197,
7783,
37174,
7,
36,
8,
198,
197,
17772,
361,
479,
27,
15,
198,
197,
197,
7783,
13841,
74,
8,
1635,
13841,
47,
8,
198,
197,
17772,
198,
197,
197,
361,
318,
10745,
6269,
7,
47,
8,
198,
197,
197,
197,
7783,
37174,
7,
36,
8,
198,
197,
197,
17772,
198,
197,
197,
197,
7783,
4808,
9435,
1082,
7,
74,
11,
350,
8,
198,
197,
197,
437,
198,
197,
437,
198,
437,
198,
198,
2,
2163,
1635,
7,
74,
3712,
46541,
11,
350,
3712,
30639,
10257,
291,
12727,
8,
198,
2,
220,
197,
7783,
479,
1635,
350,
198,
2,
886,
198,
198,
8818,
4808,
9435,
1082,
7,
76,
3712,
46541,
11,
350,
3712,
30639,
10257,
291,
12727,
8,
198,
220,
220,
220,
279,
15,
796,
350,
198,
220,
220,
220,
329,
275,
287,
40806,
2024,
13,
14781,
7,
29993,
2024,
13,
50188,
7,
12894,
896,
7,
5317,
23,
11,
285,
11,
2779,
28,
17,
36911,
352,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
357,
65,
6624,
657,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
279,
15,
796,
4808,
23352,
7,
79,
15,
8,
198,
220,
220,
220,
220,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
279,
15,
796,
350,
1343,
4808,
23352,
7,
79,
15,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
279,
15,
198,
437,
198,
198,
29113,
29113,
4242,
2235,
198,
2,
4935,
425,
3090,
1099,
329,
10073,
775,
959,
2536,
562,
23759,
198,
29113,
29113,
4242,
2235,
198,
198,
2,
350,
796,
357,
87,
16,
11,
331,
16,
11,
1976,
16,
8,
198,
2,
1195,
796,
357,
87,
17,
11,
331,
17,
11,
1976,
17,
8,
198,
2,
350,
1343,
1195,
796,
357,
87,
18,
11,
331,
18,
11,
1976,
18,
8,
198,
2,
611,
350,
14512,
1195,
788,
198,
2,
2124,
18,
796,
357,
87,
17,
1976,
16,
532,
2124,
16,
1976,
17,
8,
685,
357,
88,
17,
1976,
16,
532,
331,
16,
1976,
17,
8,
61,
17,
1976,
16,
1976,
17,
532,
357,
87,
17,
1976,
16,
532,
2124,
16,
1976,
17,
8,
61,
17,
357,
87,
17,
1976,
16,
1343,
2124,
16,
1976,
17,
8,
2361,
198,
2,
331,
18,
796,
357,
88,
17,
1976,
16,
532,
331,
16,
1976,
17,
8,
685,
357,
87,
17,
1976,
16,
532,
2124,
16,
1976,
17,
8,
61,
17,
357,
87,
17,
1976,
16,
1343,
362,
2124,
16,
1976,
17,
8,
532,
357,
88,
17,
1976,
16,
532,
331,
16,
1976,
17,
8,
61,
17,
1976,
16,
1976,
17,
2361,
532,
357,
87,
17,
1976,
16,
532,
2124,
16,
1976,
17,
8,
61,
18,
331,
16,
1976,
17,
198,
2,
1976,
18,
796,
357,
87,
17,
1976,
16,
532,
2124,
16,
1976,
17,
8,
61,
18,
1976,
16,
1976,
17,
198,
2,
611,
350,
796,
1195,
788,
198,
2,
2124,
18,
796,
362,
331,
16,
1976,
16,
685,
357,
64,
1976,
16,
61,
17,
1343,
513,
2124,
16,
61,
17,
8,
61,
17,
532,
807,
2124,
16,
331,
16,
61,
17,
1976,
16,
2361,
198,
2,
331,
18,
796,
357,
64,
1976,
16,
61,
17,
1343,
513,
2124,
16,
61,
17,
1267,
685,
1105,
2124,
16,
331,
16,
61,
17,
1976,
16,
532,
257,
61,
17,
357,
89,
16,
61,
17,
1343,
513,
2124,
16,
61,
17,
8,
61,
17,
2361,
532,
807,
331,
16,
61,
19,
1976,
16,
61,
17,
198,
2,
1976,
18,
796,
357,
17,
331,
16,
1976,
16,
8,
61,
18,
198,
198,
2,
1212,
2163,
318,
691,
284,
307,
973,
351,
7310,
2173,
319,
262,
976,
1790,
775,
959,
2536,
562,
12133,
198,
2,
87,
15255,
318,
2124,
17,
1976,
16,
532,
2124,
16,
1976,
17,
11,
290,
331,
15255,
318,
331,
17,
1976,
16,
532,
331,
16,
1976,
17,
198,
8818,
4808,
16302,
425,
62,
2860,
62,
710,
80,
7,
47,
3712,
30639,
10257,
291,
12727,
90,
51,
5512,
1195,
3712,
30639,
10257,
291,
12727,
90,
51,
5512,
2124,
15255,
3712,
51,
11,
331,
15255,
3712,
51,
8,
810,
309,
198,
197,
87,
16,
11,
331,
16,
11,
1976,
16,
796,
22715,
7,
47,
8,
198,
197,
87,
17,
11,
331,
17,
11,
1976,
17,
796,
22715,
7,
48,
8,
198,
197,
87,
15255,
17,
796,
2124,
15255,
61,
17,
198,
197,
87,
15255,
18,
796,
2124,
15255,
17,
1635,
2124,
15255,
198,
197,
5173,
316,
17,
796,
331,
15255,
61,
17,
198,
197,
89,
16,
89,
17,
796,
1976,
16,
1635,
1976,
17,
198,
197,
2,
732,
714,
3613,
257,
1178,
15082,
3736,
287,
644,
5679,
198,
197,
87,
18,
796,
2124,
15255,
1635,
357,
331,
15255,
17,
1635,
1976,
16,
89,
17,
532,
2124,
15255,
17,
1635,
357,
87,
17,
1635,
1976,
16,
1343,
2124,
16,
1635,
1976,
17,
8,
1267,
198,
197,
88,
18,
796,
331,
15255,
1635,
357,
2124,
15255,
17,
1635,
357,
87,
17,
1635,
1976,
16,
1343,
362,
1635,
2124,
16,
1635,
1976,
17,
8,
532,
331,
15255,
17,
1635,
1976,
16,
89,
17,
1267,
532,
2124,
15255,
18,
1635,
331,
16,
1635,
1976,
17,
198,
197,
89,
18,
796,
2124,
15255,
18,
1635,
1976,
16,
89,
17,
198,
197,
411,
796,
6252,
7,
87,
18,
11,
331,
18,
11,
1976,
18,
11,
2779,
62,
22019,
303,
7,
47,
4008,
198,
197,
2,
31,
30493,
318,
12102,
7,
411,
8,
1303,
12807,
414,
2198,
198,
197,
7783,
581,
198,
437,
198,
198,
2,
1212,
2163,
318,
691,
284,
307,
973,
351,
257,
966,
319,
257,
1790,
775,
959,
2536,
562,
12133,
198,
8818,
4808,
16302,
425,
62,
67,
2436,
7,
47,
3712,
30639,
10257,
291,
12727,
90,
51,
30072,
810,
309,
198,
197,
87,
11,
331,
11,
1976,
796,
22715,
7,
47,
8,
198,
197,
62,
11,
4808,
11,
4808,
11,
257,
11,
275,
796,
257,
62,
16340,
2743,
1187,
7,
8692,
62,
22019,
303,
7,
47,
4008,
198,
197,
31412,
796,
257,
1635,
1976,
61,
17,
1343,
513,
1635,
2124,
61,
17,
198,
197,
31412,
17,
796,
5766,
61,
17,
198,
197,
45579,
796,
331,
1635,
1976,
198,
197,
5431,
17,
89,
796,
2124,
1635,
331,
1635,
331,
89,
198,
197,
2,
732,
714,
3613,
757,
257,
1178,
15082,
3736,
287,
644,
5679,
198,
197,
87,
35505,
796,
362,
1635,
331,
89,
1635,
357,
5766,
17,
532,
807,
1635,
2124,
88,
17,
89,
1267,
198,
197,
88,
35505,
796,
5766,
1635,
357,
1105,
1635,
2124,
88,
17,
89,
532,
5766,
17,
1267,
532,
807,
1635,
357,
45579,
8,
61,
17,
1635,
331,
61,
17,
198,
197,
89,
35505,
796,
807,
1635,
357,
45579,
8,
61,
18,
198,
197,
411,
796,
6252,
7,
87,
35505,
11,
331,
35505,
11,
1976,
35505,
11,
2779,
62,
22019,
303,
7,
47,
4008,
198,
197,
2,
31,
30493,
318,
12102,
7,
411,
8,
1303,
12807,
414,
2198,
198,
197,
7783,
581,
198,
437,
198,
198,
2,
1212,
2163,
318,
691,
284,
307,
973,
351,
734,
2173,
319,
262,
976,
1790,
775,
959,
2536,
562,
12133,
198,
2,
7293,
1133,
2124,
15255,
290,
331,
15255,
11,
611,
484,
389,
1111,
6632,
467,
284,
4808,
16302,
425,
62,
67,
2436,
198,
8818,
1628,
425,
62,
2860,
7,
47,
3712,
30639,
10257,
291,
12727,
90,
51,
5512,
1195,
3712,
30639,
10257,
291,
12727,
90,
51,
30072,
810,
309,
198,
197,
87,
16,
11,
331,
16,
11,
1976,
16,
796,
22715,
7,
47,
8,
198,
197,
87,
17,
11,
331,
17,
11,
1976,
17,
796,
22715,
7,
48,
8,
198,
197,
87,
15255,
796,
2124,
17,
1635,
1976,
16,
532,
2124,
16,
1635,
1976,
17,
198,
197,
5173,
316,
796,
331,
17,
1635,
1976,
16,
532,
331,
16,
1635,
1976,
17,
198,
197,
361,
14808,
87,
15255,
6624,
657,
8,
1222,
357,
5173,
316,
6624,
657,
4008,
198,
197,
197,
7783,
4808,
16302,
425,
62,
67,
2436,
7,
47,
8,
198,
197,
17772,
198,
197,
197,
7783,
4808,
16302,
425,
62,
2860,
62,
710,
80,
7,
47,
11,
1195,
11,
2124,
15255,
11,
331,
15255,
8,
198,
197,
437,
198,
437,
198,
198,
2,
4342,
356,
7048,
410,
318,
257,
3967,
18253,
290,
350,
3160,
319,
257,
1790,
775,
959,
2536,
562,
12133,
198,
8818,
4808,
16302,
425,
62,
1416,
282,
283,
62,
76,
377,
7,
47,
3712,
30639,
10257,
291,
12727,
11,
410,
3712,
46541,
8,
198,
220,
220,
220,
350,
15,
796,
350,
198,
220,
220,
220,
329,
275,
287,
40806,
2024,
13,
14781,
7,
29993,
2024,
13,
50188,
7,
12894,
896,
7,
5317,
23,
11,
410,
11,
2779,
28,
17,
36911,
352,
8,
198,
220,
220,
220,
220,
220,
220,
220,
350,
15,
796,
4808,
16302,
425,
62,
67,
2436,
7,
47,
15,
8,
198,
220,
220,
220,
220,
220,
220,
220,
611,
357,
65,
6624,
352,
8,
198,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
350,
15,
796,
1628,
425,
62,
2860,
7,
47,
15,
11,
350,
8,
198,
220,
220,
220,
220,
220,
220,
220,
886,
198,
220,
220,
220,
886,
198,
220,
220,
220,
1441,
350,
15,
198,
437,
198,
198,
8818,
1628,
425,
62,
1416,
282,
283,
62,
76,
377,
7,
47,
3712,
30639,
10257,
291,
12727,
11,
410,
3712,
46541,
8,
198,
197,
361,
410,
6624,
657,
198,
197,
197,
7783,
37174,
7,
8692,
62,
22019,
303,
7,
36,
4008,
198,
197,
17772,
361,
410,
1279,
657,
198,
197,
197,
7783,
532,
4808,
16302,
425,
62,
1416,
282,
283,
62,
76,
377,
7,
47,
11,
532,
85,
8,
198,
197,
17772,
198,
197,
197,
7783,
4808,
16302,
425,
62,
1416,
282,
283,
62,
76,
377,
7,
47,
11,
410,
8,
198,
197,
437,
198,
437,
628,
628
] | 2.338721 | 2,970 |
### A Pluto.jl notebook ###
# v0.16.1
using Markdown
using InteractiveUtils
# ╔═╡ 6f9a94bd-dd28-4110-a7ca-0ed84e9c7c3f
begin
import Pkg
# activate the shared project environment
Pkg.activate(Base.current_project())
using Interpolations
using Plots
using Dierckx
using GasChromatographySimulator
using GasChromatographyTools
using Plots
using PlutoUI
TableOfContents()
end
# ╔═╡ 0b51a792-d9b8-4c29-928f-57aaf41f1c20
plotly()
# ╔═╡ 93ba6afc-4e9a-11ec-08d9-81c0a9bc502e
md"""
# Test of the RT lock functions
The test is necessary, because of failing (infinit loop in case of opt_ipt="linear", NaN abort in case of opt_itp="spline").
"""
# ╔═╡ 51ec0223-199a-4a25-8768-cd0b7e9f864d
begin
function stretched_program(n::Float64, par::GasChromatographySimulator.Parameters)
# stretch the temperature program in 'par' by a factor 'n'
if isa(par, Array)==true
error("Select an element of the array of GC-system parameters.")
else
new_tsteps = n.*par.prog.time_steps
new_T_itp = GasChromatographySimulator.temperature_interpolation(new_tsteps, par.prog.temp_steps, par.prog.gf, par.col.L)
new_pin_itp = GasChromatographySimulator.pressure_interpolation(new_tsteps, par.prog.pin_steps)
new_pout_itp = GasChromatographySimulator.pressure_interpolation(new_tsteps, par.prog.pout_steps)
new_prog = GasChromatographySimulator.Program(new_tsteps, par.prog.temp_steps, par.prog.pin_steps, par.prog.pout_steps, par.prog.gf, par.prog.a_gf, new_T_itp, new_pin_itp, new_pout_itp)
new_par = GasChromatographySimulator.Parameters(par.col, new_prog, par.sub, par.opt)
return new_par
end
end
function initial_n(n::Float64, tR_lock::Float64, ii::Int, par::GasChromatographySimulator.Parameters)
par_n = stretched_program(n, par)
sol_n = GasChromatographySimulator.solve_system_multithreads(par_n)
tR_n = sol_n[ii].u[end][1]
if n>1
while tR_n-tR_lock<0 && n<130.0
n = n*2.0
par_n = stretched_program(n, par)
sol_n = GasChromatographySimulator.solve_system_multithreads(par_n)
tR_n = sol_n[ii].u[end][1]
end
elseif n<1
while tR_n-tR_lock>0 && n>0.01
n = n*0.5
par_n = stretched_program(n, par)
sol_n = GasChromatographySimulator.solve_system_multithreads(par_n)
tR_n = sol_n[ii].u[end][1]
end
end
if n>130.0
error("The choosen retention time for locking is to big.")
elseif n<0.01
error("The choosen retention time for locking is to small.")
else
return n
end
end
function RT_locking(par::GasChromatographySimulator.Parameters, tR_lock::Float64, tR_tol::Float64, solute_RT::String; opt_itp="linear")
# estimate the factor 'n' for the temperature program to achieve the retention time 'tR_lock' for 'solute_RT' with the GC-system defined by 'par'
if isa(par, Array)==true
error("Select an element of the array of GC-systems.")
else
# find 'solute_RT' in the substances of 'par'
name = Array{String}(undef, length(par.sub))
for i=1:length(par.sub)
name[i] = par.sub[i].name
end
ii = findfirst(name.==solute_RT)
# calculate the retention time for the original (un-stretched) program
sol₀ = GasChromatographySimulator.solving_odesystem_r(par.col, par.prog, par.sub[ii], par.opt)
tR₀ = sol₀.u[end][1]
# start value for the factor 'n'
if tR₀-tR_lock<0
n₁ = initial_n(2.0, tR_lock, ii, par)
else
n₁ = initial_n(0.5, tR_lock, ii, par)
end
# using a recursive function to estimate 'n'
n = recur_RT_locking(n₁, [1.0], [tR₀], par, tR_lock, tR_tol, ii; opt_itp=opt_itp)
end
return n
end
function recur_RT_locking(n::Float64, n_vec::Array{Float64,1}, tR_vec::Array{Float64,1}, par::GasChromatographySimulator.Parameters, tR_lock::Float64, tR_tol::Float64, ii::Int64; opt_itp="linear")
# recursive function to find the factor 'n' for the temperature program to achieve the retention time 'tR_lock' for solute index 'ii' with the GC-system defined by 'par'
# calculate the retention time with the input guess 'n'
par₁ = stretched_program(n, par)
if par.opt.odesys==true
sol₁ = GasChromatographySimulator.solving_odesystem_r(par₁.col, par₁.prog, par₁.sub[ii], par₁.opt)
tR₁ = sol₁.u[end][1]
else
sol₁ = GasChromatographySimulator.solving_migration(par₁.col, par₁.prog, par₁.sub[ii], par₁.opt)
tR₁ = sol₁.u[end]
end
if abs(tR₁-tR_lock)<tR_tol
# if retention time is less than 'tR_tol' from 'tR_lock' we found the factor 'n'
return n
else
# estimate a new factor 'new_n' by linear interpolation of the factors 'n_vec' + 'n' over the corresponding retention times
new_n_vec = sort([n_vec; n])
new_tR_vec = sort([tR_vec; tR₁])
if opt_itp=="spline"
# Dierckx.jl
if length(new_tR_vec)<4
k = length(new_tR_vec)-1
else
k = 3
end
itp = Spline1D(new_tR_vec, new_n_vec, k=k)
else # opt_itp=="linear"
# Interpolations.jl
itp = LinearInterpolation(sort([tR_vec; tR₁]), sort([n_vec; n]))
end
new_n = itp(tR_lock)
#println("new_n=$(new_n), tR₁=$(tR₁)")
# use the new factor 'new_n' and call the recursive function again
return recur_RT_locking(new_n, new_n_vec, new_tR_vec, par, tR_lock, tR_tol, ii; opt_itp=opt_itp)
end
end
md"""
## Copies of the RT lock functions
In the package GasChromatographyTools.jl the functions where adapted to solve this problem.
"""
end
# ╔═╡ 5e642f09-9a8f-4cca-b61f-b27c8433a2e5
begin
opt = GasChromatographySimulator.Options(OwrenZen5(), 1e-6, 1e-3, "inlet", true)
L = 4.0
d = 0.1e-3
df = 0.1e-6
sp = "SLB5ms" # ["Rxi17SilMS" -> ok, "SLB5ms" -> ERR, "SPB50" -> ok, "Wax" -> ok, "DB5ms" -> ok, "Rxi5MS" -> ok, "genericLB", "genericJL"]
gas = "He"
col = GasChromatographySimulator.constructor_System(L, d, df, sp, gas)
db_path = "/Users/janleppert/Documents/GitHub/Publication_GCsim/data/Databases/"
db_file = "Database_append.csv"
first_alkane = "C8"
last_alkane = "C15"
sub = GasChromatographySimulator.load_solute_database(db_path, db_file, sp, gas, [first_alkane, last_alkane], zeros(2), zeros(2))
Tst = 273.15
Tref = 150.0 + Tst
pn = 101300.0
pin = 300000.0 + pn
pout = 0.0
dimless_rate = 0.4
Theat = 1000.0
Tshift = 40.0
tMref = GasChromatographySimulator.holdup_time(Tref, pin, pout, L, d, gas)
rate = dimless_rate*30/tMref
theat = Theat/rate
Tstart = sub[1].Tchar-Tst-Tshift
ΔT = 30.0
α = -3.0
prog0 = GasChromatographySimulator.constructor_Program([0.0, theat],[Tstart, Tstart+Theat], [pin, pin],[pout, pout],[ΔT, ΔT], [0.0, 0.0], [L, L], [α, α], opt.Tcontrol, L)
par0 = GasChromatographySimulator.Parameters(col, prog0, sub, opt)
tR_lock = 12*tMref
tR_tol = 1e-3
md"""
## Settings
The following settings produce the problem:
"""
end
# ╔═╡ 3de48bb6-8eb7-4a33-9a98-d5fe3a19f6c6
md"""
## The ERROR
"""
# ╔═╡ 19d1abb6-32b6-414f-bc95-55635cbaa73a
n = RT_locking(par0, tR_lock, 1e-3, last_alkane; opt_itp="spline")
# ╔═╡ 2cad8185-4fc5-4009-98df-07a2be2133c6
md"""
## Reproducing the error by stepwise calculations
"""
# ╔═╡ 30207849-cafe-4ec6-af8d-ee7b2e2e6de0
begin
# initial simulation
sol₀ = GasChromatographySimulator.solving_odesystem_r(par0.col, par0.prog, par0.sub[2], par0.opt)
tR₀ = sol₀.u[end][1]
end
# ╔═╡ 84e7e869-0fbf-4590-a8de-28855856661f
tR₀-tR_lock
# ╔═╡ c5ace7ce-cfa3-4c15-bcc1-8b66ad1c16e9
begin
# first stretch of the program
if tR₀-tR_lock<0
n₁ = initial_n(2.0, tR_lock, 2, par0)
else
n₁ = initial_n(0.5, tR_lock, 2, par0)
end
# recur function
par₁ = stretched_program(n₁, par0)
sol₁ = GasChromatographySimulator.solving_odesystem_r(par₁.col, par₁.prog, par₁.sub[2], par₁.opt)
tR₁ = sol₁.u[end][1]
n_vec₁ = sort([1.0; n₁])
tR_vec₁ = sort([tR₀; tR₁])
# first interpolation
itp₁ = Spline1D(tR_vec₁, n_vec₁, k=1)
end
# ╔═╡ c4d53222-03b1-4ce4-a49a-690945347432
tR₁-tR_lock
# ╔═╡ 6acfbf9b-f8ac-4483-8c93-17920d0d9f0e
begin
# second stretch
n₂ = itp₁(tR_lock)
par₂ = stretched_program(n₂, par0)
sol₂ = GasChromatographySimulator.solving_odesystem_r(par₂.col, par₂.prog, par₂.sub[2], par₂.opt)
tR₂ = sol₂.u[end][1]
n_vec₂ = sort([n_vec₁; n₂])
tR_vec₂ = sort([tR_vec₁; tR₂])
itp₂ = Spline1D(tR_vec₂, n_vec₂, k=2)
p1 = plot([tR_lock, tR_lock], [0.95, 0.98], label="tR_lock")
scatter!(p1, [tR₂, tR₂], [n₂, n₂], label="2")
plot!(p1, 51.0:0.001:52.0, itp₂.(51.0:0.001:52.0), xlims=(51.0,52.0), ylims=(0.95, 0.98), label="itp₂")
p1
end
# ╔═╡ 3b31f580-efe2-4a7a-89dc-228b38f2a71e
tR₂-tR_lock
# ╔═╡ b6c5126a-3d41-4809-ab9b-d554262e0668
begin
n₃ = itp₂(tR_lock)
par₃ = stretched_program(n₃, par0)
sol₃ = GasChromatographySimulator.solving_odesystem_r(par₃.col, par₃.prog, par₃.sub[2], par₃.opt)
tR₃ = sol₃.u[end][1]
n_vec₃ = sort([n_vec₂; n₃])
tR_vec₃ = sort([tR_vec₂; tR₃])
k = length(tR_vec₃)-1
itp₃ = Spline1D(tR_vec₃, n_vec₃, k=k)
scatter!(p1, [tR₃, tR₃], [n₃, n₃], label="3")
plot!(p1, 51.0:0.001:52.0, itp₃.(51.0:0.001:52.0), label="itp₃")
end
# ╔═╡ 6c5443bc-3dab-4317-99c9-1bb32b184fbc
tR₃-tR_lock
# ╔═╡ 3b1bb187-66f9-40bd-a0a5-a3c4e1a23819
begin
n₄ = itp₃(tR_lock)
par₄ = stretched_program(n₄, par0)
sol₄ = GasChromatographySimulator.solving_odesystem_r(par₄.col, par₄.prog, par₄.sub[2], par₄.opt)
tR₄ = sol₄.u[end][1]
n_vec₄ = sort([n_vec₃; n₄])
tR_vec₄ = sort([tR_vec₃; tR₄])
itp₄ = Spline1D(tR_vec₄, n_vec₄, k=3)
scatter!(p1, [tR₄, tR₄], [n₄, n₄], label="4")
plot!(p1, 51.0:0.001:52.0, itp₄.(51.0:0.001:52.0), label="itp₄")
end
# ╔═╡ b81b2905-6756-445b-b1be-c54298df8b3f
tR₄-tR_lock
# ╔═╡ eb2d9f6a-8384-427a-a036-b4f0019d8251
md"""
The proposed settings seam to lead to jumping estimations around the searched retention time.
"""
# ╔═╡ 734bae50-12fa-4717-8446-addb963b8673
begin
n₅ = itp₄(tR_lock)
par₅ = GasChromatographyTools.stretched_program(n₅, par0)
sol₅ = GasChromatographySimulator.solving_odesystem_r(par₅.col, par₅.prog, par₅.sub[2], par₅.opt)
tR₅ = sol₅.u[end][1]
n_vec₅ = sort([n_vec₄; n₅])
tR_vec₅ = sort([tR_vec₄; tR₅])
itp₅ = Spline1D(tR_vec₅, n_vec₅, k=3)
scatter!(p1, [tR₅, tR₅], [n₅, n₅], label="5")
plot!(p1, 51.0:0.001:52.0, itp₅.(51.0:0.001:52.0), xlims=(51.45,51.55), ylims=(0.969, 0.972), label="itp₅")
end
# ╔═╡ 8de716b9-ca56-42f5-aebf-39ad467f4613
tR₅-tR_lock
# ╔═╡ dadbefbf-a107-4c89-a4ef-0eb756517c1e
begin
n₆ = itp₅(tR_lock)
par₆ = GasChromatographyTools.stretched_program(n₆, par0)
sol₆ = GasChromatographySimulator.solving_odesystem_r(par₆.col, par₆.prog, par₆.sub[2], par₆.opt)
tR₆ = sol₆.u[end][1]
n_vec₆ = sort([n_vec₅; n₆])
tR_vec₆ = sort([tR_vec₅; tR₆])
tR_vec₆.-tR_lock
itp₆ = Spline1D(tR_vec₆, n_vec₆, k=3)
scatter!(p1, [tR₆, tR₆], [n₆, n₆], label="6")
plot!(p1, 51.0:0.001:52.0, itp₆.(51.0:0.001:52.0), xlims=(51.45,51.55), ylims=(0.969, 0.972), label="itp₆")
end
# ╔═╡ 313d1431-ec39-4d1e-91fd-e025efc1f5c3
tR₆-tR_lock
# ╔═╡ fab59b1e-ba32-49d3-b0f1-564db037400c
begin
n₇ = itp₆(tR_lock)
par₇ = GasChromatographyTools.stretched_program(n₇, par0)
sol₇ = GasChromatographySimulator.solving_odesystem_r(par₇.col, par₇.prog, par₇.sub[2], par₇.opt)
tR₇ = sol₇.u[end][1]
n_vec₇ = sort([n_vec₆; n₇])
tR_vec₇ = sort([tR_vec₆; tR₇])
tR_vec₇.-tR_lock
itp₇ = Spline1D(tR_vec₇, n_vec₇, k=3)
scatter!(p1, [tR₇, tR₇], [n₇, n₇], label="7")
plot!(p1, 51.0:0.001:52.0, itp₇.(51.0:0.001:52.0), xlims=(51.47,51.49), ylims=(0.9699, 0.9701), label="itp₇")
end
# ╔═╡ 25725568-ae9a-4ab0-8f98-87fec12c867a
begin
n₈ = itp₇(tR_lock)
par₈ = GasChromatographyTools.stretched_program(n₈, par0)
sol₈ = GasChromatographySimulator.solving_odesystem_r(par₈.col, par₈.prog, par₈.sub[2], par₈.opt)
tR₈ = sol₈.u[end][1]
n_vec₈ = sort([n_vec₇; n₈])
tR_vec₈ = sort([tR_vec₇; tR₈])
tR_vec₈.-tR_lock
itp₈ = Spline1D(tR_vec₈, n_vec₈, k=3)
scatter!(p1, [tR₈, tR₈], [n₈, n₈], label="8")
plot!(p1, 51.0:0.001:52.0, itp₈.(51.0:0.001:52.0), xlims=(51.47,51.49), ylims=(0.9699, 0.9701), label="itp₈")
end
# ╔═╡ 404c1d12-5217-485e-b3a6-6f024d29b544
md"""
The estimates continue to jump around tR_lock. The last estimate is further away than the one before.
Also, there seems to be some form of discontiuity of the simulation result around tR_lock, which produces the problem in the first place.
"""
# ╔═╡ 57634f00-45d5-4cf9-94f5-76319d4e5436
begin
n₉ = itp₈(tR_lock)
par₉ = GasChromatographyTools.stretched_program(n₉, par0)
sol₉ = GasChromatographySimulator.solving_odesystem_r(par₉.col, par₉.prog, par₉.sub[2], par₉.opt)
tR₉ = sol₉.u[end][1]
n_vec₉ = sort([n_vec₈; n₉])
tR_vec₉ = sort([tR_vec₈; tR₉])
tR_vec₉.-tR_lock
itp₉ = Spline1D(tR_vec₉, n_vec₉, k=3)
scatter!(p1, [tR₉, tR₉], [n₉, n₉], label="9")
plot!(p1, 51.0:0.001:52.0, itp₉.(51.0:0.001:52.0), xlims=(51.47,51.49), ylims=(0.9699, 0.9701), label="itp₉")
end
# ╔═╡ ae803a26-c436-497a-b245-a0afec92e46f
md"""
Make simulations for a range of stretch factors ``n``.
"""
# ╔═╡ 1983258e-e84b-4f39-9ce8-0e20e78a0893
begin
nn = [0.969970, 0.969971, 0.969972, 0.969973, 0.969974, 0.969975, 0.969976, 0.969977]
ttR = Array{Float64}(undef, length(nn))
for i=1:length(nn)
pars = stretched_program(nn[i], par0)
sols = GasChromatographySimulator.solving_odesystem_r(pars.col, pars.prog, pars.sub[2], pars.opt)
ttR[i] = sols.u[end][1]
end
p2 = plot([tR_lock, tR_lock], [0.95, 0.98], label="tR_lock")
plot!(p2, ttR, nn, line=(2,:solid), markers=:square, xlims=(51.479, 51.484), ylims=(0.969969,0.96998))
end
# ╔═╡ 89cbd5c0-0df6-43d3-bd5c-d3d55d669f33
begin
nnn = 0.969974.+collect(0.0000001:0.0000001:0.0000009)
tttR = Array{Float64}(undef, length(nnn))
for i=1:length(nnn)
pars = stretched_program(nnn[i], par0)
sols = GasChromatographySimulator.solving_odesystem_r(pars.col, pars.prog, pars.sub[2], pars.opt)
tttR[i] = sols.u[end][1]
end
plot!(p2, tttR, nnn, ylims=(0.9699739,0.9699751), markers=:circle)
end
# ╔═╡ f541b210-3ef8-4003-b358-065e5c5949ad
par8 = stretched_program(nnn[8], par0)
# ╔═╡ 524b256b-0c1e-48bc-ac0b-2ee7fc1eab2b
par9 = stretched_program(nnn[9], par0)
# ╔═╡ 6d15c2a4-6d1e-4b89-b9e8-ecff004c4730
sol8 = GasChromatographySimulator.solving_odesystem_r(par8.col, par8.prog, par8.sub[2], par8.opt)
# ╔═╡ 665f302d-204b-47ac-90df-c5979350707c
sol9 = GasChromatographySimulator.solving_odesystem_r(par9.col, par9.prog, par9.sub[2], par9.opt)
# ╔═╡ 56095d71-6169-44b3-89d1-7ea7f1b6ddfb
sol8.destats
# ╔═╡ a51cd1dc-50bb-4444-a0f7-c0e4229b1257
sol9.destats
# ╔═╡ 225434d5-c753-4476-8f68-f5761a454852
sol8.retcode
# ╔═╡ 9a6f436e-e50e-4697-a1d1-3d2d43fc62fc
sol9.retcode
# ╔═╡ 8a6eccf0-8740-4d69-b96b-1248557d4c4d
begin
nnnn = sort!(rand(0.9699745:0.000000001:0.9699755, 100))
ttttR = Array{Float64}(undef, length(nnnn))
for i=1:length(nnnn)
pars = stretched_program(nnnn[i], par0)
sols = GasChromatographySimulator.solving_odesystem_r(pars.col, pars.prog, pars.sub[2], pars.opt)
ttttR[i] = sols.u[end][1]
end
plot!(p2, ttttR, nnnn, ylims=(0.9699739,0.9699756), markers=:circle)
md"""
In the range around tR_lock the solution seems to be unstable. A small change in the temperature program leads to a bigger change in the retention time. There seem to be two branches of n(tR), which does not meet.
$(embed_display(p2))
"""
end
# ╔═╡ 08a0972a-55ff-42c9-838b-1a649afe9a46
md"""
## Decreased relative tolerance
The problem is not originated in the RT_lock algorithm but in the simulation itself. For certain settings a small change (like small strectch in the program) can result in bigger changes in retention time.
"""
# ╔═╡ 2d7da55e-2711-44a4-b8b9-bda6321a4c48
begin
# decrease the relative tolerance
opt_1 = GasChromatographySimulator.Options(OwrenZen5(), 1e-6, 1e-4, "inlet", true)
par_1 = GasChromatographySimulator.Parameters(col, prog0, sub, opt_1)
# repeat the simulation from above
nnnn_1 = sort!(rand(0.9699745:0.000000001:0.9699755, 100))
ttttR_1 = Array{Float64}(undef, length(nnnn_1))
for i=1:length(nnnn_1)
pars = stretched_program(nnnn_1[i], par_1)
sols = GasChromatographySimulator.solving_odesystem_r(pars.col, pars.prog, pars.sub[2], pars.opt)
ttttR_1[i] = sols.u[end][1]
end
plot!(p2, ttttR_1, nnnn_1, ylims=(0.9699739,0.9699756), markers=:v, label="reltol=1e-4")
end
# ╔═╡ 89fa3139-1d10-4dd3-90b1-d9c0f65745c6
begin
n_1 = RT_locking(par_1, tR_lock, 1e-3, last_alkane; opt_itp="spline")
par_1_s = stretched_program(n_1, par_1)
sol_1_s = GasChromatographySimulator.solving_odesystem_r(par_1_s.col, par_1_s.prog, par_1_s.sub[2], par_1_s.opt)
tR_1_s = sol_1_s.u[end][1]
scatter!(p2, [tR_1_s, tR_1_s], [n_1, n_1], ylims=(0.9699739, n_1*1.000001))
end
# ╔═╡ e9accaac-587f-498d-ab0c-a8064f573678
begin
# simulation arround n_1
n_range = (n_1-0.0001):0.000001:(n_1+0.0001)
tR_range = Array{Float64}(undef, length(n_range))
for i=1:length(n_range)
pars = stretched_program(n_range[i], par_1)
sols = GasChromatographySimulator.solving_odesystem_r(pars.col, pars.prog, pars.sub[2], pars.opt)
tR_range[i] = sols.u[end][1]
end
plot!(p2, tR_range, n_range, ylims=(n_range[1], n_range[end]), xlims=(tR_range[1], tR_range[end]))
end
# ╔═╡ 4cd795b1-efeb-430b-a025-77d0ee9d2b1b
md"""
## Modified Functions with relative difference
"""
# ╔═╡ 0bf4903c-074d-41b8-a4e8-5834ce8659d5
function recur_RT_locking_rel(n::Float64, n_vec::Array{Float64,1}, tR_vec::Array{Float64,1}, par::GasChromatographySimulator.Parameters, tR_lock::Float64, tR_tol::Float64, ii::Int64; opt_itp="linear")
# recursive function to find the factor 'n' for the temperature program to achieve the retention time 'tR_lock' for solute index 'ii' with the GC-system defined by 'par'
# calculate the retention time with the input guess 'n'
par₁ = stretched_program(n, par)
if par.opt.odesys==true
sol₁ = GasChromatographySimulator.solving_odesystem_r(par₁.col, par₁.prog, par₁.sub[ii], par₁.opt)
tR₁ = sol₁.u[end][1]
else
sol₁ = GasChromatographySimulator.solving_migration(par₁.col, par₁.prog, par₁.sub[ii], par₁.opt)
tR₁ = sol₁.u[end]
end
if abs(tR₁-tR_lock)/tR_lock<tR_tol
# if retention time is less than 'tR_tol' from 'tR_lock' we found the factor 'n'
return n
else
# estimate a new factor 'new_n' by linear interpolation of the factors 'n_vec' + 'n' over the corresponding retention times
new_n_vec = sort([n_vec; n])
new_tR_vec = sort([tR_vec; tR₁])
if opt_itp=="spline"
# Dierckx.jl
if length(new_tR_vec)<4
k = length(new_tR_vec)-1
else
k = 3
end
itp = Spline1D(new_tR_vec, new_n_vec, k=k)
else # opt_itp=="linear"
# Interpolations.jl
itp = LinearInterpolation(sort([tR_vec; tR₁]), sort([n_vec; n]))
end
new_n = itp(tR_lock)
#println("new_n=$(new_n), tR₁=$(tR₁)")
# use the new factor 'new_n' and call the recursive function again
return recur_RT_locking_rel(new_n, new_n_vec, new_tR_vec, par, tR_lock, tR_tol, ii; opt_itp=opt_itp)
end
end
# ╔═╡ be7d3bf5-d682-40e7-8161-4243354373f8
function RT_locking_rel(par::GasChromatographySimulator.Parameters, tR_lock::Float64, tR_tol::Float64, solute_RT::String; opt_itp="linear")
# estimate the factor 'n' for the temperature program to achieve the retention time 'tR_lock' for 'solute_RT' with the GC-system defined by 'par'
if isa(par, Array)==true
error("Select an element of the array of GC-systems.")
elseif tR_tol<par.opt.reltol
error("The relative tolerance for retention time locking tR_tol is to small. Use tR_tol > par.opt.reltol ($(par.opt.reltol)).")
else
# find 'solute_RT' in the substances of 'par'
name = Array{String}(undef, length(par.sub))
for i=1:length(par.sub)
name[i] = par.sub[i].name
end
ii = findfirst(name.==solute_RT)
# calculate the retention time for the original (un-stretched) program
sol₀ = GasChromatographySimulator.solving_odesystem_r(par.col, par.prog, par.sub[ii], par.opt)
tR₀ = sol₀.u[end][1]
# start value for the factor 'n'
if tR₀-tR_lock<0
n₁ = initial_n(2.0, tR_lock, ii, par)
else
n₁ = initial_n(0.5, tR_lock, ii, par)
end
# using a recursive function to estimate 'n'
n = recur_RT_locking_rel(n₁, [1.0], [tR₀], par, tR_lock, tR_tol, ii; opt_itp=opt_itp)
end
return n
end
# ╔═╡ 8a7a24e3-3896-4012-8e50-473a70ec3a44
n_rel = RT_locking_rel(par0, tR_lock, 1e-3, last_alkane; opt_itp="spline")
# ╔═╡ 4cbd8054-d1c7-4132-ae61-ef09ce6ba9e7
par0_rel = stretched_program(n_rel, par0)
# ╔═╡ b842960f-ef61-4a70-8a17-5405ec3e5ed3
sol_rel = GasChromatographySimulator.solving_odesystem_r(par0_rel.col, par0_rel.prog, par0_rel.sub[2], par0_rel.opt)
# ╔═╡ 24269e34-fc1d-4ee2-87b6-afb6a3081596
tR_rel = sol_rel.u[end][1]
# ╔═╡ 0f7e36cb-c4a7-43b5-928f-d364b82d218b
tR_rel-tR_lock
# ╔═╡ 3bdf621e-969b-42b8-85f8-5ecd261646d3
(tR_rel-tR_lock)/tR_lock
# ╔═╡ 42a1fccd-e782-42fb-91de-18c8b33d507d
md"""
# End
"""
# ╔═╡ Cell order:
# ╠═6f9a94bd-dd28-4110-a7ca-0ed84e9c7c3f
# ╠═0b51a792-d9b8-4c29-928f-57aaf41f1c20
# ╠═93ba6afc-4e9a-11ec-08d9-81c0a9bc502e
# ╠═51ec0223-199a-4a25-8768-cd0b7e9f864d
# ╠═5e642f09-9a8f-4cca-b61f-b27c8433a2e5
# ╠═3de48bb6-8eb7-4a33-9a98-d5fe3a19f6c6
# ╠═19d1abb6-32b6-414f-bc95-55635cbaa73a
# ╠═2cad8185-4fc5-4009-98df-07a2be2133c6
# ╠═30207849-cafe-4ec6-af8d-ee7b2e2e6de0
# ╠═84e7e869-0fbf-4590-a8de-28855856661f
# ╠═c5ace7ce-cfa3-4c15-bcc1-8b66ad1c16e9
# ╠═c4d53222-03b1-4ce4-a49a-690945347432
# ╠═6acfbf9b-f8ac-4483-8c93-17920d0d9f0e
# ╠═3b31f580-efe2-4a7a-89dc-228b38f2a71e
# ╠═b6c5126a-3d41-4809-ab9b-d554262e0668
# ╠═6c5443bc-3dab-4317-99c9-1bb32b184fbc
# ╠═3b1bb187-66f9-40bd-a0a5-a3c4e1a23819
# ╠═b81b2905-6756-445b-b1be-c54298df8b3f
# ╟─eb2d9f6a-8384-427a-a036-b4f0019d8251
# ╠═734bae50-12fa-4717-8446-addb963b8673
# ╠═8de716b9-ca56-42f5-aebf-39ad467f4613
# ╠═dadbefbf-a107-4c89-a4ef-0eb756517c1e
# ╠═313d1431-ec39-4d1e-91fd-e025efc1f5c3
# ╠═fab59b1e-ba32-49d3-b0f1-564db037400c
# ╠═25725568-ae9a-4ab0-8f98-87fec12c867a
# ╠═404c1d12-5217-485e-b3a6-6f024d29b544
# ╠═57634f00-45d5-4cf9-94f5-76319d4e5436
# ╠═ae803a26-c436-497a-b245-a0afec92e46f
# ╠═1983258e-e84b-4f39-9ce8-0e20e78a0893
# ╠═89cbd5c0-0df6-43d3-bd5c-d3d55d669f33
# ╠═f541b210-3ef8-4003-b358-065e5c5949ad
# ╠═524b256b-0c1e-48bc-ac0b-2ee7fc1eab2b
# ╠═6d15c2a4-6d1e-4b89-b9e8-ecff004c4730
# ╠═665f302d-204b-47ac-90df-c5979350707c
# ╠═56095d71-6169-44b3-89d1-7ea7f1b6ddfb
# ╠═a51cd1dc-50bb-4444-a0f7-c0e4229b1257
# ╠═225434d5-c753-4476-8f68-f5761a454852
# ╠═9a6f436e-e50e-4697-a1d1-3d2d43fc62fc
# ╠═8a6eccf0-8740-4d69-b96b-1248557d4c4d
# ╟─08a0972a-55ff-42c9-838b-1a649afe9a46
# ╠═2d7da55e-2711-44a4-b8b9-bda6321a4c48
# ╠═89fa3139-1d10-4dd3-90b1-d9c0f65745c6
# ╠═e9accaac-587f-498d-ab0c-a8064f573678
# ╠═4cd795b1-efeb-430b-a025-77d0ee9d2b1b
# ╠═be7d3bf5-d682-40e7-8161-4243354373f8
# ╠═0bf4903c-074d-41b8-a4e8-5834ce8659d5
# ╠═8a7a24e3-3896-4012-8e50-473a70ec3a44
# ╠═4cbd8054-d1c7-4132-ae61-ef09ce6ba9e7
# ╠═b842960f-ef61-4a70-8a17-5405ec3e5ed3
# ╠═24269e34-fc1d-4ee2-87b6-afb6a3081596
# ╠═0f7e36cb-c4a7-43b5-928f-d364b82d218b
# ╠═3bdf621e-969b-42b8-85f8-5ecd261646d3
# ╠═42a1fccd-e782-42fb-91de-18c8b33d507d
| [
21017,
317,
32217,
13,
20362,
20922,
44386,
198,
2,
410,
15,
13,
1433,
13,
16,
198,
198,
3500,
2940,
2902,
198,
3500,
21365,
18274,
4487,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
718,
69,
24,
64,
5824,
17457,
12,
1860,
2078,
12,
3901,
940,
12,
64,
22,
6888,
12,
15,
276,
5705,
68,
24,
66,
22,
66,
18,
69,
198,
27471,
198,
197,
11748,
350,
10025,
198,
220,
220,
220,
1303,
15155,
262,
4888,
1628,
2858,
198,
220,
220,
220,
350,
10025,
13,
39022,
7,
14881,
13,
14421,
62,
16302,
28955,
198,
197,
3500,
4225,
16104,
602,
198,
197,
3500,
1345,
1747,
198,
197,
3500,
360,
959,
694,
87,
198,
197,
3500,
14345,
1925,
398,
45501,
8890,
8927,
198,
197,
3500,
14345,
1925,
398,
45501,
33637,
198,
197,
3500,
1345,
1747,
198,
197,
3500,
32217,
10080,
198,
197,
10962,
5189,
15842,
3419,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
657,
65,
4349,
64,
48156,
12,
67,
24,
65,
23,
12,
19,
66,
1959,
12,
24,
2078,
69,
12,
3553,
64,
1878,
3901,
69,
16,
66,
1238,
198,
29487,
306,
3419,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
10261,
7012,
21,
1878,
66,
12,
19,
68,
24,
64,
12,
1157,
721,
12,
2919,
67,
24,
12,
6659,
66,
15,
64,
24,
15630,
35126,
68,
198,
9132,
37811,
198,
2,
6208,
286,
262,
11923,
5793,
5499,
198,
464,
1332,
318,
3306,
11,
780,
286,
9894,
357,
10745,
15003,
9052,
287,
1339,
286,
2172,
62,
10257,
2625,
29127,
1600,
11013,
45,
15614,
287,
1339,
286,
2172,
62,
270,
79,
2625,
22018,
500,
11074,
198,
37811,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
6885,
721,
15,
22047,
12,
19104,
64,
12,
19,
64,
1495,
12,
5774,
3104,
12,
10210,
15,
65,
22,
68,
24,
69,
39570,
67,
198,
27471,
198,
197,
8818,
19110,
62,
23065,
7,
77,
3712,
43879,
2414,
11,
1582,
3712,
39699,
1925,
398,
45501,
8890,
8927,
13,
48944,
8,
198,
197,
197,
2,
7539,
262,
5951,
1430,
287,
705,
1845,
6,
416,
257,
5766,
705,
77,
6,
198,
197,
197,
361,
318,
64,
7,
1845,
11,
15690,
8,
855,
7942,
198,
197,
197,
197,
18224,
7203,
17563,
281,
5002,
286,
262,
7177,
286,
20145,
12,
10057,
10007,
19570,
198,
197,
197,
17772,
198,
197,
197,
197,
3605,
62,
83,
20214,
796,
299,
15885,
1845,
13,
1676,
70,
13,
2435,
62,
20214,
198,
197,
197,
197,
3605,
62,
51,
62,
270,
79,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
11498,
21069,
62,
3849,
16104,
341,
7,
3605,
62,
83,
20214,
11,
1582,
13,
1676,
70,
13,
29510,
62,
20214,
11,
1582,
13,
1676,
70,
13,
70,
69,
11,
1582,
13,
4033,
13,
43,
8,
198,
197,
197,
197,
3605,
62,
11635,
62,
270,
79,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
36151,
62,
3849,
16104,
341,
7,
3605,
62,
83,
20214,
11,
1582,
13,
1676,
70,
13,
11635,
62,
20214,
8,
198,
197,
197,
197,
3605,
62,
79,
448,
62,
270,
79,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
36151,
62,
3849,
16104,
341,
7,
3605,
62,
83,
20214,
11,
1582,
13,
1676,
70,
13,
79,
448,
62,
20214,
8,
198,
197,
197,
197,
3605,
62,
1676,
70,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
15167,
7,
3605,
62,
83,
20214,
11,
1582,
13,
1676,
70,
13,
29510,
62,
20214,
11,
1582,
13,
1676,
70,
13,
11635,
62,
20214,
11,
1582,
13,
1676,
70,
13,
79,
448,
62,
20214,
11,
1582,
13,
1676,
70,
13,
70,
69,
11,
1582,
13,
1676,
70,
13,
64,
62,
70,
69,
11,
649,
62,
51,
62,
270,
79,
11,
649,
62,
11635,
62,
270,
79,
11,
649,
62,
79,
448,
62,
270,
79,
8,
198,
197,
197,
197,
3605,
62,
1845,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
48944,
7,
1845,
13,
4033,
11,
649,
62,
1676,
70,
11,
1582,
13,
7266,
11,
1582,
13,
8738,
8,
198,
197,
197,
197,
7783,
649,
62,
1845,
198,
197,
197,
437,
197,
198,
197,
437,
628,
197,
8818,
4238,
62,
77,
7,
77,
3712,
43879,
2414,
11,
256,
49,
62,
5354,
3712,
43879,
2414,
11,
21065,
3712,
5317,
11,
1582,
3712,
39699,
1925,
398,
45501,
8890,
8927,
13,
48944,
8,
198,
197,
220,
220,
220,
1582,
62,
77,
796,
19110,
62,
23065,
7,
77,
11,
1582,
8,
198,
197,
220,
220,
220,
1540,
62,
77,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
6442,
62,
10057,
62,
16680,
342,
40779,
7,
1845,
62,
77,
8,
198,
197,
220,
220,
220,
256,
49,
62,
77,
796,
1540,
62,
77,
58,
4178,
4083,
84,
58,
437,
7131,
16,
60,
198,
197,
220,
220,
220,
611,
299,
29,
16,
198,
197,
220,
220,
220,
220,
220,
220,
220,
981,
256,
49,
62,
77,
12,
83,
49,
62,
5354,
27,
15,
11405,
299,
27,
12952,
13,
15,
198,
197,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
299,
796,
299,
9,
17,
13,
15,
198,
197,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1582,
62,
77,
796,
19110,
62,
23065,
7,
77,
11,
1582,
8,
198,
197,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1540,
62,
77,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
6442,
62,
10057,
62,
16680,
342,
40779,
7,
1845,
62,
77,
8,
198,
197,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
256,
49,
62,
77,
796,
1540,
62,
77,
58,
4178,
4083,
84,
58,
437,
7131,
16,
60,
198,
197,
220,
220,
220,
220,
220,
220,
220,
886,
198,
197,
220,
220,
220,
2073,
361,
299,
27,
16,
198,
197,
220,
220,
220,
220,
220,
220,
220,
981,
256,
49,
62,
77,
12,
83,
49,
62,
5354,
29,
15,
11405,
299,
29,
15,
13,
486,
198,
197,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
299,
796,
299,
9,
15,
13,
20,
198,
197,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1582,
62,
77,
796,
19110,
62,
23065,
7,
77,
11,
1582,
8,
198,
197,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
1540,
62,
77,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
6442,
62,
10057,
62,
16680,
342,
40779,
7,
1845,
62,
77,
8,
198,
197,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
220,
256,
49,
62,
77,
796,
1540,
62,
77,
58,
4178,
4083,
84,
58,
437,
7131,
16,
60,
198,
197,
220,
220,
220,
220,
220,
220,
220,
886,
198,
197,
220,
220,
220,
886,
198,
197,
220,
220,
220,
611,
299,
29,
12952,
13,
15,
198,
197,
220,
220,
220,
220,
220,
220,
220,
4049,
7203,
464,
1727,
5233,
21545,
640,
329,
22656,
318,
284,
1263,
19570,
198,
197,
220,
220,
220,
2073,
361,
299,
27,
15,
13,
486,
198,
197,
220,
220,
220,
220,
220,
220,
220,
4049,
7203,
464,
1727,
5233,
21545,
640,
329,
22656,
318,
284,
1402,
19570,
198,
197,
220,
220,
220,
2073,
198,
197,
220,
220,
220,
220,
220,
220,
220,
1441,
299,
198,
197,
220,
220,
220,
886,
198,
197,
437,
628,
197,
8818,
11923,
62,
48331,
7,
1845,
3712,
39699,
1925,
398,
45501,
8890,
8927,
13,
48944,
11,
256,
49,
62,
5354,
3712,
43879,
2414,
11,
256,
49,
62,
83,
349,
3712,
43879,
2414,
11,
1540,
1133,
62,
14181,
3712,
10100,
26,
2172,
62,
270,
79,
2625,
29127,
4943,
198,
197,
197,
2,
8636,
262,
5766,
705,
77,
6,
329,
262,
5951,
1430,
284,
4620,
262,
21545,
640,
705,
83,
49,
62,
5354,
6,
329,
705,
82,
3552,
62,
14181,
6,
351,
262,
20145,
12,
10057,
5447,
416,
705,
1845,
6,
220,
198,
197,
197,
361,
318,
64,
7,
1845,
11,
15690,
8,
855,
7942,
198,
197,
197,
197,
18224,
7203,
17563,
281,
5002,
286,
262,
7177,
286,
20145,
12,
10057,
82,
19570,
198,
197,
197,
17772,
198,
197,
197,
197,
2,
1064,
705,
82,
3552,
62,
14181,
6,
287,
262,
15938,
286,
705,
1845,
6,
198,
197,
197,
197,
3672,
796,
15690,
90,
10100,
92,
7,
917,
891,
11,
4129,
7,
1845,
13,
7266,
4008,
198,
197,
197,
197,
1640,
1312,
28,
16,
25,
13664,
7,
1845,
13,
7266,
8,
198,
197,
197,
197,
197,
3672,
58,
72,
60,
796,
1582,
13,
7266,
58,
72,
4083,
3672,
198,
197,
197,
197,
437,
198,
197,
197,
197,
4178,
796,
1064,
11085,
7,
3672,
13,
855,
82,
3552,
62,
14181,
8,
198,
197,
197,
197,
2,
15284,
262,
21545,
640,
329,
262,
2656,
357,
403,
12,
49729,
8,
1430,
220,
198,
197,
197,
197,
34453,
158,
224,
222,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
13,
4033,
11,
1582,
13,
1676,
70,
11,
1582,
13,
7266,
58,
4178,
4357,
1582,
13,
8738,
8,
198,
197,
197,
197,
83,
49,
158,
224,
222,
796,
1540,
158,
224,
222,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
197,
197,
2,
923,
1988,
329,
262,
5766,
705,
77,
6,
198,
197,
197,
197,
361,
256,
49,
158,
224,
222,
12,
83,
49,
62,
5354,
27,
15,
198,
197,
197,
197,
197,
77,
158,
224,
223,
796,
4238,
62,
77,
7,
17,
13,
15,
11,
256,
49,
62,
5354,
11,
21065,
11,
1582,
8,
198,
197,
197,
197,
17772,
198,
197,
197,
197,
197,
77,
158,
224,
223,
796,
4238,
62,
77,
7,
15,
13,
20,
11,
256,
49,
62,
5354,
11,
21065,
11,
1582,
8,
198,
197,
197,
197,
437,
198,
197,
197,
197,
2,
1262,
257,
45115,
2163,
284,
8636,
705,
77,
6,
198,
197,
197,
197,
77,
796,
664,
333,
62,
14181,
62,
48331,
7,
77,
158,
224,
223,
11,
685,
16,
13,
15,
4357,
685,
83,
49,
158,
224,
222,
4357,
1582,
11,
256,
49,
62,
5354,
11,
256,
49,
62,
83,
349,
11,
21065,
26,
2172,
62,
270,
79,
28,
8738,
62,
270,
79,
8,
198,
197,
197,
437,
198,
197,
197,
7783,
299,
197,
197,
198,
197,
437,
628,
197,
8818,
664,
333,
62,
14181,
62,
48331,
7,
77,
3712,
43879,
2414,
11,
299,
62,
35138,
3712,
19182,
90,
43879,
2414,
11,
16,
5512,
256,
49,
62,
35138,
3712,
19182,
90,
43879,
2414,
11,
16,
5512,
1582,
3712,
39699,
1925,
398,
45501,
8890,
8927,
13,
48944,
11,
256,
49,
62,
5354,
3712,
43879,
2414,
11,
256,
49,
62,
83,
349,
3712,
43879,
2414,
11,
21065,
3712,
5317,
2414,
26,
2172,
62,
270,
79,
2625,
29127,
4943,
198,
197,
197,
2,
45115,
2163,
284,
1064,
262,
5766,
705,
77,
6,
329,
262,
5951,
1430,
284,
4620,
262,
21545,
640,
705,
83,
49,
62,
5354,
6,
329,
1540,
1133,
6376,
705,
4178,
6,
351,
262,
20145,
12,
10057,
5447,
416,
705,
1845,
6,
198,
197,
197,
2,
15284,
262,
21545,
640,
351,
262,
5128,
4724,
705,
77,
6,
198,
197,
197,
1845,
158,
224,
223,
796,
19110,
62,
23065,
7,
77,
11,
1582,
8,
198,
197,
197,
361,
1582,
13,
8738,
13,
4147,
893,
855,
7942,
198,
197,
197,
197,
34453,
158,
224,
223,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
158,
224,
223,
13,
4033,
11,
1582,
158,
224,
223,
13,
1676,
70,
11,
1582,
158,
224,
223,
13,
7266,
58,
4178,
4357,
1582,
158,
224,
223,
13,
8738,
8,
198,
197,
197,
197,
83,
49,
158,
224,
223,
796,
1540,
158,
224,
223,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
197,
17772,
198,
197,
197,
197,
34453,
158,
224,
223,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
76,
4254,
7,
1845,
158,
224,
223,
13,
4033,
11,
1582,
158,
224,
223,
13,
1676,
70,
11,
1582,
158,
224,
223,
13,
7266,
58,
4178,
4357,
1582,
158,
224,
223,
13,
8738,
8,
198,
197,
197,
197,
83,
49,
158,
224,
223,
796,
1540,
158,
224,
223,
13,
84,
58,
437,
60,
198,
197,
197,
437,
198,
197,
197,
361,
2352,
7,
83,
49,
158,
224,
223,
12,
83,
49,
62,
5354,
8,
27,
83,
49,
62,
83,
349,
198,
197,
197,
197,
2,
611,
21545,
640,
318,
1342,
621,
705,
83,
49,
62,
83,
349,
6,
422,
705,
83,
49,
62,
5354,
6,
356,
1043,
262,
5766,
705,
77,
6,
198,
197,
197,
197,
7783,
299,
198,
197,
197,
17772,
198,
197,
197,
197,
2,
8636,
257,
649,
5766,
705,
3605,
62,
77,
6,
416,
14174,
39555,
341,
286,
262,
5087,
705,
77,
62,
35138,
6,
1343,
705,
77,
6,
625,
262,
11188,
21545,
1661,
198,
197,
197,
197,
3605,
62,
77,
62,
35138,
796,
3297,
26933,
77,
62,
35138,
26,
299,
12962,
198,
197,
197,
197,
3605,
62,
83,
49,
62,
35138,
796,
3297,
26933,
83,
49,
62,
35138,
26,
256,
49,
158,
224,
223,
12962,
198,
197,
197,
197,
361,
2172,
62,
270,
79,
855,
1,
22018,
500,
1,
198,
197,
197,
197,
197,
2,
360,
959,
694,
87,
13,
20362,
198,
197,
197,
197,
197,
361,
4129,
7,
3605,
62,
83,
49,
62,
35138,
8,
27,
19,
198,
197,
197,
197,
197,
197,
74,
796,
4129,
7,
3605,
62,
83,
49,
62,
35138,
13219,
16,
198,
197,
197,
197,
197,
17772,
198,
197,
197,
197,
197,
197,
74,
796,
513,
198,
197,
197,
197,
197,
437,
198,
197,
197,
197,
197,
270,
79,
796,
13341,
500,
16,
35,
7,
3605,
62,
83,
49,
62,
35138,
11,
649,
62,
77,
62,
35138,
11,
479,
28,
74,
8,
198,
197,
197,
197,
17772,
1303,
2172,
62,
270,
79,
855,
1,
29127,
1,
198,
197,
197,
197,
197,
2,
4225,
16104,
602,
13,
20362,
198,
197,
197,
197,
197,
270,
79,
796,
44800,
9492,
16104,
341,
7,
30619,
26933,
83,
49,
62,
35138,
26,
256,
49,
158,
224,
223,
46570,
3297,
26933,
77,
62,
35138,
26,
299,
60,
4008,
198,
197,
197,
197,
437,
198,
197,
197,
197,
3605,
62,
77,
796,
340,
79,
7,
83,
49,
62,
5354,
8,
198,
197,
197,
197,
2,
35235,
7203,
3605,
62,
77,
43641,
7,
3605,
62,
77,
828,
256,
49,
158,
224,
223,
43641,
7,
83,
49,
158,
224,
223,
8,
4943,
198,
197,
197,
197,
2,
779,
262,
649,
5766,
705,
3605,
62,
77,
6,
290,
869,
262,
45115,
2163,
757,
198,
197,
197,
197,
7783,
664,
333,
62,
14181,
62,
48331,
7,
3605,
62,
77,
11,
649,
62,
77,
62,
35138,
11,
649,
62,
83,
49,
62,
35138,
11,
1582,
11,
256,
49,
62,
5354,
11,
256,
49,
62,
83,
349,
11,
21065,
26,
2172,
62,
270,
79,
28,
8738,
62,
270,
79,
8,
198,
197,
197,
437,
198,
197,
437,
198,
197,
198,
197,
9132,
37811,
198,
197,
2235,
6955,
444,
286,
262,
11923,
5793,
5499,
198,
197,
818,
262,
5301,
14345,
1925,
398,
45501,
33637,
13,
20362,
262,
5499,
810,
16573,
284,
8494,
428,
1917,
13,
198,
197,
37811,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
642,
68,
41290,
69,
2931,
12,
24,
64,
23,
69,
12,
19,
13227,
12,
65,
5333,
69,
12,
65,
1983,
66,
5705,
2091,
64,
17,
68,
20,
198,
27471,
198,
197,
8738,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
29046,
7,
46,
86,
918,
47573,
20,
22784,
352,
68,
12,
21,
11,
352,
68,
12,
18,
11,
366,
259,
1616,
1600,
2081,
8,
198,
197,
43,
796,
604,
13,
15,
198,
197,
67,
796,
657,
13,
16,
68,
12,
18,
198,
197,
7568,
796,
657,
13,
16,
68,
12,
21,
198,
197,
2777,
796,
366,
8634,
33,
20,
907,
1,
1303,
14631,
49,
29992,
1558,
15086,
5653,
1,
4613,
12876,
11,
366,
8634,
33,
20,
907,
1,
4613,
13793,
49,
11,
366,
4303,
33,
1120,
1,
4613,
12876,
11,
366,
54,
897,
1,
4613,
12876,
11,
366,
11012,
20,
907,
1,
4613,
12876,
11,
366,
49,
29992,
20,
5653,
1,
4613,
12876,
11,
366,
41357,
30501,
1600,
366,
41357,
41,
43,
8973,
198,
197,
22649,
796,
366,
1544,
1,
198,
197,
4033,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
41571,
273,
62,
11964,
7,
43,
11,
288,
11,
47764,
11,
599,
11,
3623,
8,
628,
197,
9945,
62,
6978,
796,
12813,
14490,
14,
13881,
293,
381,
861,
14,
38354,
14,
38,
270,
16066,
14,
15202,
341,
62,
15916,
14323,
14,
7890,
14,
27354,
18826,
30487,
220,
198,
197,
9945,
62,
7753,
796,
366,
38105,
62,
33295,
13,
40664,
1,
198,
197,
11085,
62,
971,
1531,
796,
366,
34,
23,
1,
198,
197,
12957,
62,
971,
1531,
796,
366,
34,
1314,
1,
198,
197,
7266,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
2220,
62,
82,
3552,
62,
48806,
7,
9945,
62,
6978,
11,
20613,
62,
7753,
11,
599,
11,
3623,
11,
685,
11085,
62,
971,
1531,
11,
938,
62,
971,
1531,
4357,
1976,
27498,
7,
17,
828,
1976,
27498,
7,
17,
4008,
198,
197,
198,
197,
51,
301,
796,
38549,
13,
1314,
198,
197,
51,
5420,
796,
6640,
13,
15,
1343,
309,
301,
198,
197,
21999,
796,
8949,
6200,
13,
15,
198,
197,
11635,
796,
5867,
830,
13,
15,
1343,
279,
77,
198,
197,
79,
448,
796,
657,
13,
15,
198,
197,
27740,
1203,
62,
4873,
796,
657,
13,
19,
198,
197,
464,
265,
796,
8576,
13,
15,
198,
197,
51,
30846,
796,
2319,
13,
15,
198,
197,
83,
44,
5420,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
2946,
929,
62,
2435,
7,
51,
5420,
11,
6757,
11,
279,
448,
11,
406,
11,
288,
11,
3623,
8,
198,
197,
4873,
796,
5391,
1203,
62,
4873,
9,
1270,
14,
83,
44,
5420,
198,
197,
1169,
265,
796,
383,
265,
14,
4873,
198,
197,
51,
9688,
796,
850,
58,
16,
4083,
51,
10641,
12,
51,
301,
12,
51,
30846,
198,
197,
198,
197,
138,
242,
51,
796,
1542,
13,
15,
198,
197,
17394,
796,
532,
18,
13,
15,
198,
197,
1676,
70,
15,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
41571,
273,
62,
15167,
26933,
15,
13,
15,
11,
23776,
38430,
51,
9688,
11,
309,
9688,
10,
464,
265,
4357,
685,
11635,
11,
6757,
38430,
79,
448,
11,
279,
448,
38430,
138,
242,
51,
11,
37455,
51,
4357,
685,
15,
13,
15,
11,
657,
13,
15,
4357,
685,
43,
11,
406,
4357,
685,
17394,
11,
26367,
4357,
2172,
13,
51,
13716,
11,
406,
8,
198,
197,
198,
197,
1845,
15,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
48944,
7,
4033,
11,
1172,
15,
11,
850,
11,
2172,
8,
628,
197,
83,
49,
62,
5354,
796,
1105,
9,
83,
44,
5420,
198,
197,
83,
49,
62,
83,
349,
796,
352,
68,
12,
18,
198,
197,
9132,
37811,
198,
197,
2235,
16163,
198,
197,
464,
1708,
6460,
4439,
262,
1917,
25,
198,
197,
37811,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
513,
2934,
2780,
11848,
21,
12,
23,
1765,
22,
12,
19,
64,
2091,
12,
24,
64,
4089,
12,
67,
20,
5036,
18,
64,
1129,
69,
21,
66,
21,
198,
9132,
37811,
198,
2235,
383,
33854,
198,
37811,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
678,
67,
16,
6485,
21,
12,
2624,
65,
21,
12,
37309,
69,
12,
15630,
3865,
12,
37864,
2327,
66,
7012,
64,
4790,
64,
198,
77,
796,
11923,
62,
48331,
7,
1845,
15,
11,
256,
49,
62,
5354,
11,
352,
68,
12,
18,
11,
938,
62,
971,
1531,
26,
2172,
62,
270,
79,
2625,
22018,
500,
4943,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
362,
66,
324,
23,
21652,
12,
19,
16072,
20,
12,
7029,
24,
12,
4089,
7568,
12,
2998,
64,
17,
1350,
17,
16945,
66,
21,
198,
9132,
37811,
198,
2235,
36551,
2259,
262,
4049,
416,
2239,
3083,
16765,
198,
37811,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
1542,
1238,
3695,
2920,
12,
66,
8635,
12,
19,
721,
21,
12,
1878,
23,
67,
12,
1453,
22,
65,
17,
68,
17,
68,
21,
2934,
15,
198,
27471,
198,
197,
2,
4238,
18640,
198,
197,
34453,
158,
224,
222,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
15,
13,
4033,
11,
1582,
15,
13,
1676,
70,
11,
1582,
15,
13,
7266,
58,
17,
4357,
1582,
15,
13,
8738,
8,
198,
197,
83,
49,
158,
224,
222,
796,
1540,
158,
224,
222,
13,
84,
58,
437,
7131,
16,
60,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
9508,
68,
22,
68,
23,
3388,
12,
15,
69,
19881,
12,
2231,
3829,
12,
64,
23,
2934,
12,
25270,
2816,
5332,
2791,
5333,
69,
198,
83,
49,
158,
224,
222,
12,
83,
49,
62,
5354,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
269,
20,
558,
22,
344,
12,
12993,
64,
18,
12,
19,
66,
1314,
12,
65,
535,
16,
12,
23,
65,
2791,
324,
16,
66,
1433,
68,
24,
198,
27471,
198,
197,
2,
717,
7539,
286,
262,
1430,
198,
197,
361,
256,
49,
158,
224,
222,
12,
83,
49,
62,
5354,
27,
15,
198,
197,
220,
220,
220,
299,
158,
224,
223,
796,
4238,
62,
77,
7,
17,
13,
15,
11,
256,
49,
62,
5354,
11,
362,
11,
1582,
15,
8,
198,
197,
17772,
198,
197,
220,
220,
220,
299,
158,
224,
223,
796,
4238,
62,
77,
7,
15,
13,
20,
11,
256,
49,
62,
5354,
11,
362,
11,
1582,
15,
8,
198,
197,
437,
198,
197,
2,
664,
333,
2163,
198,
197,
1845,
158,
224,
223,
796,
19110,
62,
23065,
7,
77,
158,
224,
223,
11,
1582,
15,
8,
198,
197,
34453,
158,
224,
223,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
158,
224,
223,
13,
4033,
11,
1582,
158,
224,
223,
13,
1676,
70,
11,
1582,
158,
224,
223,
13,
7266,
58,
17,
4357,
1582,
158,
224,
223,
13,
8738,
8,
198,
197,
83,
49,
158,
224,
223,
796,
1540,
158,
224,
223,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
77,
62,
35138,
158,
224,
223,
796,
3297,
26933,
16,
13,
15,
26,
299,
158,
224,
223,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
223,
796,
3297,
26933,
83,
49,
158,
224,
222,
26,
256,
49,
158,
224,
223,
12962,
198,
197,
2,
717,
39555,
341,
198,
197,
270,
79,
158,
224,
223,
796,
13341,
500,
16,
35,
7,
83,
49,
62,
35138,
158,
224,
223,
11,
299,
62,
35138,
158,
224,
223,
11,
479,
28,
16,
8,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
269,
19,
67,
4310,
23148,
12,
3070,
65,
16,
12,
19,
344,
19,
12,
64,
2920,
64,
12,
3388,
2931,
2231,
2682,
4524,
2624,
198,
83,
49,
158,
224,
223,
12,
83,
49,
62,
5354,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
718,
330,
69,
19881,
24,
65,
12,
69,
23,
330,
12,
2598,
5999,
12,
23,
66,
6052,
12,
21738,
1238,
67,
15,
67,
24,
69,
15,
68,
198,
27471,
198,
197,
2,
1218,
7539,
220,
198,
197,
77,
158,
224,
224,
796,
340,
79,
158,
224,
223,
7,
83,
49,
62,
5354,
8,
198,
197,
1845,
158,
224,
224,
796,
19110,
62,
23065,
7,
77,
158,
224,
224,
11,
1582,
15,
8,
198,
197,
34453,
158,
224,
224,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
158,
224,
224,
13,
4033,
11,
1582,
158,
224,
224,
13,
1676,
70,
11,
1582,
158,
224,
224,
13,
7266,
58,
17,
4357,
1582,
158,
224,
224,
13,
8738,
8,
198,
197,
83,
49,
158,
224,
224,
796,
1540,
158,
224,
224,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
77,
62,
35138,
158,
224,
224,
796,
3297,
26933,
77,
62,
35138,
158,
224,
223,
26,
299,
158,
224,
224,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
224,
796,
3297,
26933,
83,
49,
62,
35138,
158,
224,
223,
26,
256,
49,
158,
224,
224,
12962,
198,
197,
270,
79,
158,
224,
224,
796,
13341,
500,
16,
35,
7,
83,
49,
62,
35138,
158,
224,
224,
11,
299,
62,
35138,
158,
224,
224,
11,
479,
28,
17,
8,
628,
197,
79,
16,
796,
7110,
26933,
83,
49,
62,
5354,
11,
256,
49,
62,
5354,
4357,
685,
15,
13,
3865,
11,
657,
13,
4089,
4357,
6167,
2625,
83,
49,
62,
5354,
4943,
198,
197,
1416,
1436,
0,
7,
79,
16,
11,
685,
83,
49,
158,
224,
224,
11,
256,
49,
158,
224,
224,
4357,
685,
77,
158,
224,
224,
11,
299,
158,
224,
224,
4357,
6167,
2625,
17,
4943,
198,
197,
29487,
0,
7,
79,
16,
11,
6885,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
11,
340,
79,
158,
224,
224,
12195,
4349,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
828,
2124,
2475,
82,
16193,
4349,
13,
15,
11,
4309,
13,
15,
828,
331,
2475,
82,
16193,
15,
13,
3865,
11,
657,
13,
4089,
828,
6167,
2625,
270,
79,
158,
224,
224,
4943,
198,
197,
79,
16,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
513,
65,
3132,
69,
39322,
12,
22521,
17,
12,
19,
64,
22,
64,
12,
4531,
17896,
12,
23815,
65,
2548,
69,
17,
64,
4869,
68,
198,
83,
49,
158,
224,
224,
12,
83,
49,
62,
5354,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
275,
21,
66,
20,
19420,
64,
12,
18,
67,
3901,
12,
22148,
24,
12,
397,
24,
65,
12,
67,
44218,
29119,
68,
15,
35809,
198,
27471,
198,
197,
77,
158,
224,
225,
796,
340,
79,
158,
224,
224,
7,
83,
49,
62,
5354,
8,
198,
197,
1845,
158,
224,
225,
796,
19110,
62,
23065,
7,
77,
158,
224,
225,
11,
1582,
15,
8,
198,
197,
34453,
158,
224,
225,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
158,
224,
225,
13,
4033,
11,
1582,
158,
224,
225,
13,
1676,
70,
11,
1582,
158,
224,
225,
13,
7266,
58,
17,
4357,
1582,
158,
224,
225,
13,
8738,
8,
198,
197,
83,
49,
158,
224,
225,
796,
1540,
158,
224,
225,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
77,
62,
35138,
158,
224,
225,
796,
3297,
26933,
77,
62,
35138,
158,
224,
224,
26,
299,
158,
224,
225,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
225,
796,
3297,
26933,
83,
49,
62,
35138,
158,
224,
224,
26,
256,
49,
158,
224,
225,
12962,
198,
197,
74,
796,
4129,
7,
83,
49,
62,
35138,
158,
224,
225,
13219,
16,
198,
197,
270,
79,
158,
224,
225,
796,
13341,
500,
16,
35,
7,
83,
49,
62,
35138,
158,
224,
225,
11,
299,
62,
35138,
158,
224,
225,
11,
479,
28,
74,
8,
198,
197,
198,
197,
1416,
1436,
0,
7,
79,
16,
11,
685,
83,
49,
158,
224,
225,
11,
256,
49,
158,
224,
225,
4357,
685,
77,
158,
224,
225,
11,
299,
158,
224,
225,
4357,
6167,
2625,
18,
4943,
198,
197,
29487,
0,
7,
79,
16,
11,
6885,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
11,
340,
79,
158,
224,
225,
12195,
4349,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
828,
6167,
2625,
270,
79,
158,
224,
225,
4943,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
718,
66,
20,
34938,
15630,
12,
18,
67,
397,
12,
3559,
1558,
12,
2079,
66,
24,
12,
16,
11848,
2624,
65,
22883,
69,
15630,
198,
83,
49,
158,
224,
225,
12,
83,
49,
62,
5354,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
513,
65,
16,
11848,
23451,
12,
2791,
69,
24,
12,
1821,
17457,
12,
64,
15,
64,
20,
12,
64,
18,
66,
19,
68,
16,
64,
23721,
1129,
198,
27471,
198,
197,
77,
158,
224,
226,
796,
340,
79,
158,
224,
225,
7,
83,
49,
62,
5354,
8,
198,
197,
1845,
158,
224,
226,
796,
19110,
62,
23065,
7,
77,
158,
224,
226,
11,
1582,
15,
8,
198,
197,
34453,
158,
224,
226,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
158,
224,
226,
13,
4033,
11,
1582,
158,
224,
226,
13,
1676,
70,
11,
1582,
158,
224,
226,
13,
7266,
58,
17,
4357,
1582,
158,
224,
226,
13,
8738,
8,
198,
197,
83,
49,
158,
224,
226,
796,
1540,
158,
224,
226,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
77,
62,
35138,
158,
224,
226,
796,
3297,
26933,
77,
62,
35138,
158,
224,
225,
26,
299,
158,
224,
226,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
226,
796,
3297,
26933,
83,
49,
62,
35138,
158,
224,
225,
26,
256,
49,
158,
224,
226,
12962,
198,
197,
270,
79,
158,
224,
226,
796,
13341,
500,
16,
35,
7,
83,
49,
62,
35138,
158,
224,
226,
11,
299,
62,
35138,
158,
224,
226,
11,
479,
28,
18,
8,
198,
197,
198,
197,
1416,
1436,
0,
7,
79,
16,
11,
685,
83,
49,
158,
224,
226,
11,
256,
49,
158,
224,
226,
4357,
685,
77,
158,
224,
226,
11,
299,
158,
224,
226,
4357,
6167,
2625,
19,
4943,
198,
197,
29487,
0,
7,
79,
16,
11,
6885,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
11,
340,
79,
158,
224,
226,
12195,
4349,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
828,
6167,
2625,
270,
79,
158,
224,
226,
4943,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
275,
6659,
65,
1959,
2713,
12,
21,
38219,
12,
43489,
65,
12,
65,
16,
1350,
12,
66,
4051,
27728,
7568,
23,
65,
18,
69,
198,
83,
49,
158,
224,
226,
12,
83,
49,
62,
5354,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
36649,
17,
67,
24,
69,
21,
64,
12,
23,
22842,
12,
42363,
64,
12,
64,
48597,
12,
65,
19,
69,
405,
1129,
67,
23,
28072,
198,
9132,
37811,
198,
464,
5150,
6460,
15787,
284,
1085,
284,
14284,
3959,
602,
1088,
262,
16499,
21545,
640,
13,
198,
37811,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
767,
2682,
65,
3609,
1120,
12,
1065,
13331,
12,
2857,
1558,
12,
23,
27260,
12,
2860,
65,
4846,
18,
65,
23,
45758,
198,
27471,
220,
198,
197,
77,
158,
224,
227,
796,
340,
79,
158,
224,
226,
7,
83,
49,
62,
5354,
8,
198,
197,
1845,
158,
224,
227,
796,
14345,
1925,
398,
45501,
33637,
13,
49729,
62,
23065,
7,
77,
158,
224,
227,
11,
1582,
15,
8,
198,
197,
34453,
158,
224,
227,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
158,
224,
227,
13,
4033,
11,
1582,
158,
224,
227,
13,
1676,
70,
11,
1582,
158,
224,
227,
13,
7266,
58,
17,
4357,
1582,
158,
224,
227,
13,
8738,
8,
198,
197,
83,
49,
158,
224,
227,
796,
1540,
158,
224,
227,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
77,
62,
35138,
158,
224,
227,
796,
3297,
26933,
77,
62,
35138,
158,
224,
226,
26,
299,
158,
224,
227,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
227,
796,
3297,
26933,
83,
49,
62,
35138,
158,
224,
226,
26,
256,
49,
158,
224,
227,
12962,
198,
197,
270,
79,
158,
224,
227,
796,
13341,
500,
16,
35,
7,
83,
49,
62,
35138,
158,
224,
227,
11,
299,
62,
35138,
158,
224,
227,
11,
479,
28,
18,
8,
628,
197,
1416,
1436,
0,
7,
79,
16,
11,
685,
83,
49,
158,
224,
227,
11,
256,
49,
158,
224,
227,
4357,
685,
77,
158,
224,
227,
11,
299,
158,
224,
227,
4357,
6167,
2625,
20,
4943,
198,
197,
29487,
0,
7,
79,
16,
11,
6885,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
11,
340,
79,
158,
224,
227,
12195,
4349,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
828,
2124,
2475,
82,
16193,
4349,
13,
2231,
11,
4349,
13,
2816,
828,
331,
2475,
82,
16193,
15,
13,
38819,
11,
657,
13,
24,
4761,
828,
6167,
2625,
270,
79,
158,
224,
227,
4943,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
807,
2934,
22,
1433,
65,
24,
12,
6888,
3980,
12,
3682,
69,
20,
12,
64,
1765,
69,
12,
2670,
324,
24669,
69,
3510,
1485,
198,
83,
49,
158,
224,
227,
12,
83,
49,
62,
5354,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
9955,
65,
891,
19881,
12,
64,
15982,
12,
19,
66,
4531,
12,
64,
19,
891,
12,
15,
1765,
2425,
2996,
1558,
66,
16,
68,
198,
27471,
198,
197,
77,
158,
224,
228,
796,
340,
79,
158,
224,
227,
7,
83,
49,
62,
5354,
8,
198,
197,
1845,
158,
224,
228,
796,
14345,
1925,
398,
45501,
33637,
13,
49729,
62,
23065,
7,
77,
158,
224,
228,
11,
1582,
15,
8,
198,
197,
34453,
158,
224,
228,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
158,
224,
228,
13,
4033,
11,
1582,
158,
224,
228,
13,
1676,
70,
11,
1582,
158,
224,
228,
13,
7266,
58,
17,
4357,
1582,
158,
224,
228,
13,
8738,
8,
198,
197,
83,
49,
158,
224,
228,
796,
1540,
158,
224,
228,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
77,
62,
35138,
158,
224,
228,
796,
3297,
26933,
77,
62,
35138,
158,
224,
227,
26,
299,
158,
224,
228,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
228,
796,
3297,
26933,
83,
49,
62,
35138,
158,
224,
227,
26,
256,
49,
158,
224,
228,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
228,
7874,
83,
49,
62,
5354,
198,
197,
270,
79,
158,
224,
228,
796,
13341,
500,
16,
35,
7,
83,
49,
62,
35138,
158,
224,
228,
11,
299,
62,
35138,
158,
224,
228,
11,
479,
28,
18,
8,
628,
197,
1416,
1436,
0,
7,
79,
16,
11,
685,
83,
49,
158,
224,
228,
11,
256,
49,
158,
224,
228,
4357,
685,
77,
158,
224,
228,
11,
299,
158,
224,
228,
4357,
6167,
2625,
21,
4943,
198,
197,
29487,
0,
7,
79,
16,
11,
6885,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
11,
340,
79,
158,
224,
228,
12195,
4349,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
828,
2124,
2475,
82,
16193,
4349,
13,
2231,
11,
4349,
13,
2816,
828,
331,
2475,
82,
16193,
15,
13,
38819,
11,
657,
13,
24,
4761,
828,
6167,
2625,
270,
79,
158,
224,
228,
4943,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
35897,
67,
1415,
3132,
12,
721,
2670,
12,
19,
67,
16,
68,
12,
6420,
16344,
12,
68,
36629,
891,
66,
16,
69,
20,
66,
18,
198,
83,
49,
158,
224,
228,
12,
83,
49,
62,
5354,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
7843,
3270,
65,
16,
68,
12,
7012,
2624,
12,
2920,
67,
18,
12,
65,
15,
69,
16,
12,
20,
2414,
9945,
15,
2718,
7029,
66,
198,
27471,
198,
197,
77,
158,
224,
229,
796,
340,
79,
158,
224,
228,
7,
83,
49,
62,
5354,
8,
198,
197,
1845,
158,
224,
229,
796,
14345,
1925,
398,
45501,
33637,
13,
49729,
62,
23065,
7,
77,
158,
224,
229,
11,
1582,
15,
8,
198,
197,
34453,
158,
224,
229,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
158,
224,
229,
13,
4033,
11,
1582,
158,
224,
229,
13,
1676,
70,
11,
1582,
158,
224,
229,
13,
7266,
58,
17,
4357,
1582,
158,
224,
229,
13,
8738,
8,
198,
197,
83,
49,
158,
224,
229,
796,
1540,
158,
224,
229,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
77,
62,
35138,
158,
224,
229,
796,
3297,
26933,
77,
62,
35138,
158,
224,
228,
26,
299,
158,
224,
229,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
229,
796,
3297,
26933,
83,
49,
62,
35138,
158,
224,
228,
26,
256,
49,
158,
224,
229,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
229,
7874,
83,
49,
62,
5354,
198,
197,
270,
79,
158,
224,
229,
796,
13341,
500,
16,
35,
7,
83,
49,
62,
35138,
158,
224,
229,
11,
299,
62,
35138,
158,
224,
229,
11,
479,
28,
18,
8,
628,
197,
1416,
1436,
0,
7,
79,
16,
11,
685,
83,
49,
158,
224,
229,
11,
256,
49,
158,
224,
229,
4357,
685,
77,
158,
224,
229,
11,
299,
158,
224,
229,
4357,
6167,
2625,
22,
4943,
198,
197,
29487,
0,
7,
79,
16,
11,
6885,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
11,
340,
79,
158,
224,
229,
12195,
4349,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
828,
2124,
2475,
82,
16193,
4349,
13,
2857,
11,
4349,
13,
2920,
828,
331,
2475,
82,
16193,
15,
13,
4846,
2079,
11,
657,
13,
5607,
486,
828,
6167,
2625,
270,
79,
158,
224,
229,
4943,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
36100,
13381,
3104,
12,
3609,
24,
64,
12,
19,
397,
15,
12,
23,
69,
4089,
12,
5774,
69,
721,
1065,
66,
23,
3134,
64,
198,
27471,
198,
197,
77,
158,
224,
230,
796,
340,
79,
158,
224,
229,
7,
83,
49,
62,
5354,
8,
198,
197,
1845,
158,
224,
230,
796,
14345,
1925,
398,
45501,
33637,
13,
49729,
62,
23065,
7,
77,
158,
224,
230,
11,
1582,
15,
8,
198,
197,
34453,
158,
224,
230,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
158,
224,
230,
13,
4033,
11,
1582,
158,
224,
230,
13,
1676,
70,
11,
1582,
158,
224,
230,
13,
7266,
58,
17,
4357,
1582,
158,
224,
230,
13,
8738,
8,
198,
197,
83,
49,
158,
224,
230,
796,
1540,
158,
224,
230,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
77,
62,
35138,
158,
224,
230,
796,
3297,
26933,
77,
62,
35138,
158,
224,
229,
26,
299,
158,
224,
230,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
230,
796,
3297,
26933,
83,
49,
62,
35138,
158,
224,
229,
26,
256,
49,
158,
224,
230,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
230,
7874,
83,
49,
62,
5354,
198,
197,
270,
79,
158,
224,
230,
796,
13341,
500,
16,
35,
7,
83,
49,
62,
35138,
158,
224,
230,
11,
299,
62,
35138,
158,
224,
230,
11,
479,
28,
18,
8,
628,
197,
1416,
1436,
0,
7,
79,
16,
11,
685,
83,
49,
158,
224,
230,
11,
256,
49,
158,
224,
230,
4357,
685,
77,
158,
224,
230,
11,
299,
158,
224,
230,
4357,
6167,
2625,
23,
4943,
198,
197,
29487,
0,
7,
79,
16,
11,
6885,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
11,
340,
79,
158,
224,
230,
12195,
4349,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
828,
2124,
2475,
82,
16193,
4349,
13,
2857,
11,
4349,
13,
2920,
828,
331,
2475,
82,
16193,
15,
13,
4846,
2079,
11,
657,
13,
5607,
486,
828,
6167,
2625,
270,
79,
158,
224,
230,
4943,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
32320,
66,
16,
67,
1065,
12,
4309,
1558,
12,
32642,
68,
12,
65,
18,
64,
21,
12,
21,
69,
40839,
67,
1959,
65,
47576,
198,
9132,
37811,
198,
464,
7746,
2555,
284,
4391,
1088,
256,
49,
62,
5354,
13,
383,
938,
8636,
318,
2252,
1497,
621,
262,
530,
878,
13,
198,
198,
7583,
11,
612,
2331,
284,
307,
617,
1296,
286,
16141,
72,
14834,
286,
262,
18640,
1255,
1088,
256,
49,
62,
5354,
11,
543,
11073,
262,
1917,
287,
262,
717,
1295,
13,
220,
198,
37811,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
642,
4304,
2682,
69,
405,
12,
2231,
67,
20,
12,
19,
12993,
24,
12,
5824,
69,
20,
12,
4304,
35175,
67,
19,
68,
4051,
2623,
198,
27471,
198,
197,
77,
158,
224,
231,
796,
340,
79,
158,
224,
230,
7,
83,
49,
62,
5354,
8,
198,
197,
1845,
158,
224,
231,
796,
14345,
1925,
398,
45501,
33637,
13,
49729,
62,
23065,
7,
77,
158,
224,
231,
11,
1582,
15,
8,
198,
197,
34453,
158,
224,
231,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
158,
224,
231,
13,
4033,
11,
1582,
158,
224,
231,
13,
1676,
70,
11,
1582,
158,
224,
231,
13,
7266,
58,
17,
4357,
1582,
158,
224,
231,
13,
8738,
8,
198,
197,
83,
49,
158,
224,
231,
796,
1540,
158,
224,
231,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
77,
62,
35138,
158,
224,
231,
796,
3297,
26933,
77,
62,
35138,
158,
224,
230,
26,
299,
158,
224,
231,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
231,
796,
3297,
26933,
83,
49,
62,
35138,
158,
224,
230,
26,
256,
49,
158,
224,
231,
12962,
198,
197,
83,
49,
62,
35138,
158,
224,
231,
7874,
83,
49,
62,
5354,
198,
197,
270,
79,
158,
224,
231,
796,
13341,
500,
16,
35,
7,
83,
49,
62,
35138,
158,
224,
231,
11,
299,
62,
35138,
158,
224,
231,
11,
479,
28,
18,
8,
628,
197,
1416,
1436,
0,
7,
79,
16,
11,
685,
83,
49,
158,
224,
231,
11,
256,
49,
158,
224,
231,
4357,
685,
77,
158,
224,
231,
11,
299,
158,
224,
231,
4357,
6167,
2625,
24,
4943,
198,
197,
29487,
0,
7,
79,
16,
11,
6885,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
11,
340,
79,
158,
224,
231,
12195,
4349,
13,
15,
25,
15,
13,
8298,
25,
4309,
13,
15,
828,
2124,
2475,
82,
16193,
4349,
13,
2857,
11,
4349,
13,
2920,
828,
331,
2475,
82,
16193,
15,
13,
4846,
2079,
11,
657,
13,
5607,
486,
828,
6167,
2625,
270,
79,
158,
224,
231,
4943,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
257,
68,
43564,
64,
2075,
12,
66,
43690,
12,
38073,
64,
12,
65,
22995,
12,
64,
15,
1878,
721,
5892,
68,
3510,
69,
198,
9132,
37811,
198,
12050,
27785,
329,
257,
2837,
286,
7539,
5087,
7559,
77,
15506,
13,
198,
37811,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
13540,
25600,
68,
12,
68,
5705,
65,
12,
19,
69,
2670,
12,
24,
344,
23,
12,
15,
68,
1238,
68,
3695,
64,
2919,
6052,
198,
27471,
198,
197,
20471,
796,
685,
15,
13,
4846,
2079,
2154,
11,
657,
13,
4846,
2079,
4869,
11,
657,
13,
4846,
2079,
4761,
11,
657,
13,
4846,
2079,
4790,
11,
657,
13,
4846,
2079,
4524,
11,
657,
13,
4846,
2079,
2425,
11,
657,
13,
4846,
2079,
4304,
11,
657,
13,
4846,
2079,
3324,
60,
198,
197,
926,
49,
796,
15690,
90,
43879,
2414,
92,
7,
917,
891,
11,
4129,
7,
20471,
4008,
198,
197,
1640,
1312,
28,
16,
25,
13664,
7,
20471,
8,
198,
197,
220,
220,
220,
13544,
796,
19110,
62,
23065,
7,
20471,
58,
72,
4357,
1582,
15,
8,
198,
197,
220,
220,
220,
1540,
82,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
79,
945,
13,
4033,
11,
13544,
13,
1676,
70,
11,
13544,
13,
7266,
58,
17,
4357,
13544,
13,
8738,
8,
198,
197,
220,
220,
220,
256,
83,
49,
58,
72,
60,
796,
1540,
82,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
437,
198,
197,
79,
17,
796,
7110,
26933,
83,
49,
62,
5354,
11,
256,
49,
62,
5354,
4357,
685,
15,
13,
3865,
11,
657,
13,
4089,
4357,
6167,
2625,
83,
49,
62,
5354,
4943,
198,
197,
29487,
0,
7,
79,
17,
11,
256,
83,
49,
11,
299,
77,
11,
1627,
16193,
17,
11,
25,
39390,
828,
19736,
28,
25,
23415,
11,
2124,
2475,
82,
16193,
4349,
13,
31714,
11,
6885,
13,
34137,
828,
331,
2475,
82,
16193,
15,
13,
4846,
2079,
3388,
11,
15,
13,
4846,
34808,
4008,
197,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
9919,
66,
17457,
20,
66,
15,
12,
15,
7568,
21,
12,
3559,
67,
18,
12,
17457,
20,
66,
12,
67,
18,
67,
2816,
67,
36657,
69,
2091,
198,
27471,
198,
197,
20471,
77,
796,
657,
13,
4846,
2079,
4524,
13,
10,
33327,
7,
15,
13,
2388,
8298,
25,
15,
13,
2388,
8298,
25,
15,
13,
10535,
24,
8,
198,
197,
926,
83,
49,
796,
15690,
90,
43879,
2414,
92,
7,
917,
891,
11,
4129,
7,
20471,
77,
4008,
198,
197,
1640,
1312,
28,
16,
25,
13664,
7,
20471,
77,
8,
198,
197,
220,
220,
220,
13544,
796,
19110,
62,
23065,
7,
20471,
77,
58,
72,
4357,
1582,
15,
8,
198,
197,
220,
220,
220,
1540,
82,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
79,
945,
13,
4033,
11,
13544,
13,
1676,
70,
11,
13544,
13,
7266,
58,
17,
4357,
13544,
13,
8738,
8,
198,
197,
220,
220,
220,
256,
926,
49,
58,
72,
60,
796,
1540,
82,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
437,
198,
197,
29487,
0,
7,
79,
17,
11,
256,
926,
49,
11,
299,
20471,
11,
331,
2475,
82,
16193,
15,
13,
4846,
39647,
2670,
11,
15,
13,
4846,
2079,
48365,
828,
19736,
28,
25,
45597,
8,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
277,
20,
3901,
65,
21536,
12,
18,
891,
23,
12,
7029,
18,
12,
65,
31128,
12,
15,
2996,
68,
20,
66,
3270,
2920,
324,
198,
1845,
23,
796,
19110,
62,
23065,
7,
20471,
77,
58,
23,
4357,
1582,
15,
8,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
642,
1731,
65,
11645,
65,
12,
15,
66,
16,
68,
12,
2780,
15630,
12,
330,
15,
65,
12,
17,
1453,
22,
16072,
16,
68,
397,
17,
65,
198,
1845,
24,
796,
19110,
62,
23065,
7,
20471,
77,
58,
24,
4357,
1582,
15,
8,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
718,
67,
1314,
66,
17,
64,
19,
12,
21,
67,
16,
68,
12,
19,
65,
4531,
12,
65,
24,
68,
23,
12,
721,
487,
22914,
66,
2857,
1270,
198,
34453,
23,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
23,
13,
4033,
11,
1582,
23,
13,
1676,
70,
11,
1582,
23,
13,
7266,
58,
17,
4357,
1582,
23,
13,
8738,
8,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
718,
2996,
69,
22709,
67,
12,
18638,
65,
12,
2857,
330,
12,
3829,
7568,
12,
66,
3270,
3720,
14877,
24038,
66,
198,
34453,
24,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
24,
13,
4033,
11,
1582,
24,
13,
1676,
70,
11,
1582,
24,
13,
7266,
58,
17,
4357,
1582,
24,
13,
8738,
8,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
38089,
3865,
67,
4869,
12,
21,
22172,
12,
2598,
65,
18,
12,
4531,
67,
16,
12,
22,
18213,
22,
69,
16,
65,
21,
1860,
21855,
198,
34453,
23,
13,
16520,
1381,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
257,
4349,
10210,
16,
17896,
12,
1120,
11848,
12,
2598,
2598,
12,
64,
15,
69,
22,
12,
66,
15,
68,
19,
23539,
65,
1065,
3553,
198,
34453,
24,
13,
16520,
1381,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
18500,
47101,
67,
20,
12,
66,
44550,
12,
2598,
4304,
12,
23,
69,
3104,
12,
69,
3553,
5333,
64,
2231,
2780,
4309,
198,
34453,
23,
13,
1186,
8189,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
860,
64,
21,
69,
43690,
68,
12,
68,
1120,
68,
12,
19,
40035,
12,
64,
16,
67,
16,
12,
18,
67,
17,
67,
3559,
16072,
5237,
16072,
198,
34453,
24,
13,
1186,
8189,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
807,
64,
21,
68,
535,
69,
15,
12,
5774,
1821,
12,
19,
67,
3388,
12,
65,
4846,
65,
12,
1065,
2780,
41948,
67,
19,
66,
19,
67,
198,
27471,
220,
198,
197,
20471,
20471,
796,
3297,
0,
7,
25192,
7,
15,
13,
4846,
39647,
2231,
25,
15,
13,
8269,
16,
25,
15,
13,
4846,
2079,
38172,
11,
1802,
4008,
198,
197,
926,
926,
49,
796,
15690,
90,
43879,
2414,
92,
7,
917,
891,
11,
4129,
7,
20471,
20471,
4008,
198,
197,
1640,
1312,
28,
16,
25,
13664,
7,
20471,
20471,
8,
198,
197,
220,
220,
220,
13544,
796,
19110,
62,
23065,
7,
20471,
20471,
58,
72,
4357,
1582,
15,
8,
198,
197,
220,
220,
220,
1540,
82,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
79,
945,
13,
4033,
11,
13544,
13,
1676,
70,
11,
13544,
13,
7266,
58,
17,
4357,
13544,
13,
8738,
8,
198,
197,
220,
220,
220,
256,
926,
83,
49,
58,
72,
60,
796,
1540,
82,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
437,
198,
197,
29487,
0,
7,
79,
17,
11,
256,
926,
83,
49,
11,
299,
20471,
77,
11,
331,
2475,
82,
16193,
15,
13,
4846,
39647,
2670,
11,
15,
13,
4846,
2079,
38219,
828,
19736,
28,
25,
45597,
8,
198,
197,
9132,
37811,
198,
197,
818,
262,
2837,
1088,
256,
49,
62,
5354,
262,
4610,
2331,
284,
307,
21354,
13,
317,
1402,
1487,
287,
262,
5951,
1430,
5983,
284,
257,
5749,
1487,
287,
262,
21545,
640,
13,
1318,
1283,
284,
307,
734,
13737,
286,
299,
7,
83,
49,
828,
543,
857,
407,
1826,
13,
628,
197,
3,
7,
20521,
62,
13812,
7,
79,
17,
4008,
198,
197,
37811,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
8487,
64,
2931,
4761,
64,
12,
2816,
487,
12,
3682,
66,
24,
12,
23,
2548,
65,
12,
16,
64,
33300,
8635,
24,
64,
3510,
198,
9132,
37811,
198,
2235,
36400,
839,
3585,
15621,
198,
198,
464,
1917,
318,
407,
20973,
287,
262,
11923,
62,
5354,
11862,
475,
287,
262,
18640,
2346,
13,
1114,
1728,
6460,
257,
1402,
1487,
357,
2339,
1402,
1937,
310,
354,
287,
262,
1430,
8,
460,
1255,
287,
5749,
2458,
287,
21545,
640,
13,
198,
37811,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
362,
67,
22,
6814,
2816,
68,
12,
1983,
1157,
12,
2598,
64,
19,
12,
65,
23,
65,
24,
12,
43444,
5066,
2481,
64,
19,
66,
2780,
198,
27471,
198,
197,
2,
10070,
262,
3585,
15621,
198,
197,
8738,
62,
16,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
29046,
7,
46,
86,
918,
47573,
20,
22784,
352,
68,
12,
21,
11,
352,
68,
12,
19,
11,
366,
259,
1616,
1600,
2081,
8,
198,
197,
1845,
62,
16,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
48944,
7,
4033,
11,
1172,
15,
11,
850,
11,
2172,
62,
16,
8,
198,
197,
2,
9585,
262,
18640,
422,
2029,
198,
197,
20471,
20471,
62,
16,
796,
3297,
0,
7,
25192,
7,
15,
13,
4846,
39647,
2231,
25,
15,
13,
8269,
16,
25,
15,
13,
4846,
2079,
38172,
11,
1802,
4008,
198,
197,
926,
926,
49,
62,
16,
796,
15690,
90,
43879,
2414,
92,
7,
917,
891,
11,
4129,
7,
20471,
20471,
62,
16,
4008,
198,
197,
1640,
1312,
28,
16,
25,
13664,
7,
20471,
20471,
62,
16,
8,
198,
197,
220,
220,
220,
13544,
796,
19110,
62,
23065,
7,
20471,
20471,
62,
16,
58,
72,
4357,
1582,
62,
16,
8,
198,
197,
220,
220,
220,
1540,
82,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
79,
945,
13,
4033,
11,
13544,
13,
1676,
70,
11,
13544,
13,
7266,
58,
17,
4357,
13544,
13,
8738,
8,
198,
197,
220,
220,
220,
256,
926,
83,
49,
62,
16,
58,
72,
60,
796,
1540,
82,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
437,
198,
197,
29487,
0,
7,
79,
17,
11,
256,
926,
83,
49,
62,
16,
11,
299,
20471,
77,
62,
16,
11,
331,
2475,
82,
16193,
15,
13,
4846,
39647,
2670,
11,
15,
13,
4846,
2079,
38219,
828,
19736,
28,
25,
85,
11,
6167,
2625,
2411,
83,
349,
28,
16,
68,
12,
19,
4943,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
9919,
13331,
18,
20219,
12,
16,
67,
940,
12,
19,
1860,
18,
12,
3829,
65,
16,
12,
67,
24,
66,
15,
69,
37680,
2231,
66,
21,
198,
27471,
198,
197,
77,
62,
16,
796,
11923,
62,
48331,
7,
1845,
62,
16,
11,
256,
49,
62,
5354,
11,
352,
68,
12,
18,
11,
938,
62,
971,
1531,
26,
2172,
62,
270,
79,
2625,
22018,
500,
4943,
198,
197,
1845,
62,
16,
62,
82,
796,
19110,
62,
23065,
7,
77,
62,
16,
11,
1582,
62,
16,
8,
198,
197,
34453,
62,
16,
62,
82,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
62,
16,
62,
82,
13,
4033,
11,
1582,
62,
16,
62,
82,
13,
1676,
70,
11,
1582,
62,
16,
62,
82,
13,
7266,
58,
17,
4357,
1582,
62,
16,
62,
82,
13,
8738,
8,
198,
197,
83,
49,
62,
16,
62,
82,
796,
1540,
62,
16,
62,
82,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
1416,
1436,
0,
7,
79,
17,
11,
685,
83,
49,
62,
16,
62,
82,
11,
256,
49,
62,
16,
62,
82,
4357,
685,
77,
62,
16,
11,
299,
62,
16,
4357,
331,
2475,
82,
16193,
15,
13,
4846,
39647,
2670,
11,
299,
62,
16,
9,
16,
13,
2388,
486,
4008,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
304,
24,
43552,
330,
12,
44617,
69,
12,
36260,
67,
12,
397,
15,
66,
12,
64,
1795,
2414,
69,
3553,
2623,
3695,
198,
27471,
198,
197,
2,
18640,
610,
744,
299,
62,
16,
198,
197,
77,
62,
9521,
796,
357,
77,
62,
16,
12,
15,
13,
18005,
2599,
15,
13,
2388,
486,
37498,
77,
62,
16,
10,
15,
13,
18005,
8,
198,
197,
83,
49,
62,
9521,
796,
15690,
90,
43879,
2414,
92,
7,
917,
891,
11,
4129,
7,
77,
62,
9521,
4008,
198,
197,
1640,
1312,
28,
16,
25,
13664,
7,
77,
62,
9521,
8,
198,
197,
220,
220,
220,
13544,
796,
19110,
62,
23065,
7,
77,
62,
9521,
58,
72,
4357,
1582,
62,
16,
8,
198,
197,
220,
220,
220,
1540,
82,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
79,
945,
13,
4033,
11,
13544,
13,
1676,
70,
11,
13544,
13,
7266,
58,
17,
4357,
13544,
13,
8738,
8,
198,
197,
220,
220,
220,
256,
49,
62,
9521,
58,
72,
60,
796,
1540,
82,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
437,
198,
197,
29487,
0,
7,
79,
17,
11,
256,
49,
62,
9521,
11,
299,
62,
9521,
11,
331,
2475,
82,
16193,
77,
62,
9521,
58,
16,
4357,
299,
62,
9521,
58,
437,
46570,
2124,
2475,
82,
16193,
83,
49,
62,
9521,
58,
16,
4357,
256,
49,
62,
9521,
58,
437,
60,
4008,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
604,
10210,
41544,
65,
16,
12,
891,
1765,
12,
31794,
65,
12,
64,
36629,
12,
3324,
67,
15,
1453,
24,
67,
17,
65,
16,
65,
198,
9132,
37811,
198,
2235,
40499,
40480,
351,
3585,
3580,
198,
37811,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
657,
19881,
2920,
3070,
66,
12,
2998,
19,
67,
12,
3901,
65,
23,
12,
64,
19,
68,
23,
12,
3365,
2682,
344,
23,
36445,
67,
20,
198,
8818,
664,
333,
62,
14181,
62,
48331,
62,
2411,
7,
77,
3712,
43879,
2414,
11,
299,
62,
35138,
3712,
19182,
90,
43879,
2414,
11,
16,
5512,
256,
49,
62,
35138,
3712,
19182,
90,
43879,
2414,
11,
16,
5512,
1582,
3712,
39699,
1925,
398,
45501,
8890,
8927,
13,
48944,
11,
256,
49,
62,
5354,
3712,
43879,
2414,
11,
256,
49,
62,
83,
349,
3712,
43879,
2414,
11,
21065,
3712,
5317,
2414,
26,
2172,
62,
270,
79,
2625,
29127,
4943,
198,
197,
2,
45115,
2163,
284,
1064,
262,
5766,
705,
77,
6,
329,
262,
5951,
1430,
284,
4620,
262,
21545,
640,
705,
83,
49,
62,
5354,
6,
329,
1540,
1133,
6376,
705,
4178,
6,
351,
262,
20145,
12,
10057,
5447,
416,
705,
1845,
6,
198,
197,
2,
15284,
262,
21545,
640,
351,
262,
5128,
4724,
705,
77,
6,
198,
197,
1845,
158,
224,
223,
796,
19110,
62,
23065,
7,
77,
11,
1582,
8,
198,
197,
361,
1582,
13,
8738,
13,
4147,
893,
855,
7942,
198,
197,
197,
34453,
158,
224,
223,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
158,
224,
223,
13,
4033,
11,
1582,
158,
224,
223,
13,
1676,
70,
11,
1582,
158,
224,
223,
13,
7266,
58,
4178,
4357,
1582,
158,
224,
223,
13,
8738,
8,
198,
197,
197,
83,
49,
158,
224,
223,
796,
1540,
158,
224,
223,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
17772,
198,
197,
197,
34453,
158,
224,
223,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
76,
4254,
7,
1845,
158,
224,
223,
13,
4033,
11,
1582,
158,
224,
223,
13,
1676,
70,
11,
1582,
158,
224,
223,
13,
7266,
58,
4178,
4357,
1582,
158,
224,
223,
13,
8738,
8,
198,
197,
197,
83,
49,
158,
224,
223,
796,
1540,
158,
224,
223,
13,
84,
58,
437,
60,
198,
197,
437,
198,
197,
361,
2352,
7,
83,
49,
158,
224,
223,
12,
83,
49,
62,
5354,
20679,
83,
49,
62,
5354,
27,
83,
49,
62,
83,
349,
198,
197,
197,
2,
611,
21545,
640,
318,
1342,
621,
705,
83,
49,
62,
83,
349,
6,
422,
705,
83,
49,
62,
5354,
6,
356,
1043,
262,
5766,
705,
77,
6,
198,
197,
197,
7783,
299,
198,
197,
17772,
198,
197,
197,
2,
8636,
257,
649,
5766,
705,
3605,
62,
77,
6,
416,
14174,
39555,
341,
286,
262,
5087,
705,
77,
62,
35138,
6,
1343,
705,
77,
6,
625,
262,
11188,
21545,
1661,
198,
197,
197,
3605,
62,
77,
62,
35138,
796,
3297,
26933,
77,
62,
35138,
26,
299,
12962,
198,
197,
197,
3605,
62,
83,
49,
62,
35138,
796,
3297,
26933,
83,
49,
62,
35138,
26,
256,
49,
158,
224,
223,
12962,
198,
197,
197,
361,
2172,
62,
270,
79,
855,
1,
22018,
500,
1,
198,
197,
197,
197,
2,
360,
959,
694,
87,
13,
20362,
198,
197,
197,
197,
361,
4129,
7,
3605,
62,
83,
49,
62,
35138,
8,
27,
19,
198,
197,
197,
197,
197,
74,
796,
4129,
7,
3605,
62,
83,
49,
62,
35138,
13219,
16,
198,
197,
197,
197,
17772,
198,
197,
197,
197,
197,
74,
796,
513,
198,
197,
197,
197,
437,
198,
197,
197,
197,
270,
79,
796,
13341,
500,
16,
35,
7,
3605,
62,
83,
49,
62,
35138,
11,
649,
62,
77,
62,
35138,
11,
479,
28,
74,
8,
198,
197,
197,
17772,
1303,
2172,
62,
270,
79,
855,
1,
29127,
1,
198,
197,
197,
197,
2,
4225,
16104,
602,
13,
20362,
198,
197,
197,
197,
270,
79,
796,
44800,
9492,
16104,
341,
7,
30619,
26933,
83,
49,
62,
35138,
26,
256,
49,
158,
224,
223,
46570,
3297,
26933,
77,
62,
35138,
26,
299,
60,
4008,
198,
197,
197,
437,
198,
197,
197,
3605,
62,
77,
796,
340,
79,
7,
83,
49,
62,
5354,
8,
198,
197,
197,
2,
35235,
7203,
3605,
62,
77,
43641,
7,
3605,
62,
77,
828,
256,
49,
158,
224,
223,
43641,
7,
83,
49,
158,
224,
223,
8,
4943,
198,
197,
197,
2,
779,
262,
649,
5766,
705,
3605,
62,
77,
6,
290,
869,
262,
45115,
2163,
757,
198,
197,
197,
7783,
664,
333,
62,
14181,
62,
48331,
62,
2411,
7,
3605,
62,
77,
11,
649,
62,
77,
62,
35138,
11,
649,
62,
83,
49,
62,
35138,
11,
1582,
11,
256,
49,
62,
5354,
11,
256,
49,
62,
83,
349,
11,
21065,
26,
2172,
62,
270,
79,
28,
8738,
62,
270,
79,
8,
198,
197,
437,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
307,
22,
67,
18,
19881,
20,
12,
67,
43950,
12,
1821,
68,
22,
12,
23,
25948,
12,
40090,
2091,
4051,
34770,
69,
23,
198,
8818,
11923,
62,
48331,
62,
2411,
7,
1845,
3712,
39699,
1925,
398,
45501,
8890,
8927,
13,
48944,
11,
256,
49,
62,
5354,
3712,
43879,
2414,
11,
256,
49,
62,
83,
349,
3712,
43879,
2414,
11,
1540,
1133,
62,
14181,
3712,
10100,
26,
2172,
62,
270,
79,
2625,
29127,
4943,
198,
197,
2,
8636,
262,
5766,
705,
77,
6,
329,
262,
5951,
1430,
284,
4620,
262,
21545,
640,
705,
83,
49,
62,
5354,
6,
329,
705,
82,
3552,
62,
14181,
6,
351,
262,
20145,
12,
10057,
5447,
416,
705,
1845,
6,
220,
198,
197,
361,
318,
64,
7,
1845,
11,
15690,
8,
855,
7942,
198,
197,
197,
18224,
7203,
17563,
281,
5002,
286,
262,
7177,
286,
20145,
12,
10057,
82,
19570,
198,
197,
17772,
361,
256,
49,
62,
83,
349,
27,
1845,
13,
8738,
13,
2411,
83,
349,
198,
197,
197,
18224,
7203,
464,
3585,
15621,
329,
21545,
640,
22656,
256,
49,
62,
83,
349,
318,
284,
1402,
13,
5765,
256,
49,
62,
83,
349,
1875,
1582,
13,
8738,
13,
2411,
83,
349,
7198,
7,
1845,
13,
8738,
13,
2411,
83,
349,
4008,
19570,
198,
197,
17772,
198,
197,
197,
2,
1064,
705,
82,
3552,
62,
14181,
6,
287,
262,
15938,
286,
705,
1845,
6,
198,
197,
197,
3672,
796,
15690,
90,
10100,
92,
7,
917,
891,
11,
4129,
7,
1845,
13,
7266,
4008,
198,
197,
197,
1640,
1312,
28,
16,
25,
13664,
7,
1845,
13,
7266,
8,
198,
197,
197,
197,
3672,
58,
72,
60,
796,
1582,
13,
7266,
58,
72,
4083,
3672,
198,
197,
197,
437,
198,
197,
197,
4178,
796,
1064,
11085,
7,
3672,
13,
855,
82,
3552,
62,
14181,
8,
198,
197,
197,
2,
15284,
262,
21545,
640,
329,
262,
2656,
357,
403,
12,
49729,
8,
1430,
220,
198,
197,
197,
34453,
158,
224,
222,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
13,
4033,
11,
1582,
13,
1676,
70,
11,
1582,
13,
7266,
58,
4178,
4357,
1582,
13,
8738,
8,
198,
197,
197,
83,
49,
158,
224,
222,
796,
1540,
158,
224,
222,
13,
84,
58,
437,
7131,
16,
60,
198,
197,
197,
2,
923,
1988,
329,
262,
5766,
705,
77,
6,
198,
197,
197,
361,
256,
49,
158,
224,
222,
12,
83,
49,
62,
5354,
27,
15,
198,
197,
197,
197,
77,
158,
224,
223,
796,
4238,
62,
77,
7,
17,
13,
15,
11,
256,
49,
62,
5354,
11,
21065,
11,
1582,
8,
198,
197,
197,
17772,
198,
197,
197,
197,
77,
158,
224,
223,
796,
4238,
62,
77,
7,
15,
13,
20,
11,
256,
49,
62,
5354,
11,
21065,
11,
1582,
8,
198,
197,
197,
437,
198,
197,
197,
2,
1262,
257,
45115,
2163,
284,
8636,
705,
77,
6,
198,
197,
197,
77,
796,
664,
333,
62,
14181,
62,
48331,
62,
2411,
7,
77,
158,
224,
223,
11,
685,
16,
13,
15,
4357,
685,
83,
49,
158,
224,
222,
4357,
1582,
11,
256,
49,
62,
5354,
11,
256,
49,
62,
83,
349,
11,
21065,
26,
2172,
62,
270,
79,
28,
8738,
62,
270,
79,
8,
198,
197,
437,
198,
197,
7783,
299,
197,
197,
198,
437,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
807,
64,
22,
64,
1731,
68,
18,
12,
2548,
4846,
12,
21844,
17,
12,
23,
68,
1120,
12,
37804,
64,
2154,
721,
18,
64,
2598,
198,
77,
62,
2411,
796,
11923,
62,
48331,
62,
2411,
7,
1845,
15,
11,
256,
49,
62,
5354,
11,
352,
68,
12,
18,
11,
938,
62,
971,
1531,
26,
2172,
62,
270,
79,
2625,
22018,
500,
4943,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
604,
66,
17457,
1795,
4051,
12,
67,
16,
66,
22,
12,
19,
19924,
12,
3609,
5333,
12,
891,
2931,
344,
21,
7012,
24,
68,
22,
198,
1845,
15,
62,
2411,
796,
19110,
62,
23065,
7,
77,
62,
2411,
11,
1582,
15,
8,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
275,
5705,
1959,
1899,
69,
12,
891,
5333,
12,
19,
64,
2154,
12,
23,
64,
1558,
12,
20,
26598,
721,
18,
68,
20,
276,
18,
198,
34453,
62,
2411,
796,
14345,
1925,
398,
45501,
8890,
8927,
13,
82,
10890,
62,
4147,
6781,
62,
81,
7,
1845,
15,
62,
2411,
13,
4033,
11,
1582,
15,
62,
2411,
13,
1676,
70,
11,
1582,
15,
62,
2411,
13,
7266,
58,
17,
4357,
1582,
15,
62,
2411,
13,
8738,
8,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
1987,
26276,
68,
2682,
12,
16072,
16,
67,
12,
19,
1453,
17,
12,
5774,
65,
21,
12,
1878,
65,
21,
64,
21495,
1314,
4846,
198,
83,
49,
62,
2411,
796,
1540,
62,
2411,
13,
84,
58,
437,
7131,
16,
60,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
657,
69,
22,
68,
2623,
21101,
12,
66,
19,
64,
22,
12,
3559,
65,
20,
12,
24,
2078,
69,
12,
67,
26780,
65,
6469,
67,
28727,
65,
198,
83,
49,
62,
2411,
12,
83,
49,
62,
5354,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
513,
65,
7568,
21,
2481,
68,
12,
38819,
65,
12,
3682,
65,
23,
12,
5332,
69,
23,
12,
20,
21142,
2075,
1433,
3510,
67,
18,
198,
7,
83,
49,
62,
2411,
12,
83,
49,
62,
5354,
20679,
83,
49,
62,
5354,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
5433,
64,
16,
69,
535,
67,
12,
68,
46519,
12,
3682,
21855,
12,
6420,
2934,
12,
1507,
66,
23,
65,
2091,
67,
35378,
67,
198,
9132,
37811,
198,
2,
5268,
198,
37811,
198,
198,
2,
2343,
243,
242,
28670,
22880,
94,
12440,
1502,
25,
198,
2,
2343,
243,
254,
28670,
21,
69,
24,
64,
5824,
17457,
12,
1860,
2078,
12,
3901,
940,
12,
64,
22,
6888,
12,
15,
276,
5705,
68,
24,
66,
22,
66,
18,
69,
198,
2,
2343,
243,
254,
28670,
15,
65,
4349,
64,
48156,
12,
67,
24,
65,
23,
12,
19,
66,
1959,
12,
24,
2078,
69,
12,
3553,
64,
1878,
3901,
69,
16,
66,
1238,
198,
2,
2343,
243,
254,
28670,
6052,
7012,
21,
1878,
66,
12,
19,
68,
24,
64,
12,
1157,
721,
12,
2919,
67,
24,
12,
6659,
66,
15,
64,
24,
15630,
35126,
68,
198,
2,
2343,
243,
254,
28670,
4349,
721,
15,
22047,
12,
19104,
64,
12,
19,
64,
1495,
12,
5774,
3104,
12,
10210,
15,
65,
22,
68,
24,
69,
39570,
67,
198,
2,
2343,
243,
254,
28670,
20,
68,
41290,
69,
2931,
12,
24,
64,
23,
69,
12,
19,
13227,
12,
65,
5333,
69,
12,
65,
1983,
66,
5705,
2091,
64,
17,
68,
20,
198,
2,
2343,
243,
254,
28670,
18,
2934,
2780,
11848,
21,
12,
23,
1765,
22,
12,
19,
64,
2091,
12,
24,
64,
4089,
12,
67,
20,
5036,
18,
64,
1129,
69,
21,
66,
21,
198,
2,
2343,
243,
254,
28670,
1129,
67,
16,
6485,
21,
12,
2624,
65,
21,
12,
37309,
69,
12,
15630,
3865,
12,
37864,
2327,
66,
7012,
64,
4790,
64,
198,
2,
2343,
243,
254,
28670,
17,
66,
324,
23,
21652,
12,
19,
16072,
20,
12,
7029,
24,
12,
4089,
7568,
12,
2998,
64,
17,
1350,
17,
16945,
66,
21,
198,
2,
2343,
243,
254,
28670,
1270,
1238,
3695,
2920,
12,
66,
8635,
12,
19,
721,
21,
12,
1878,
23,
67,
12,
1453,
22,
65,
17,
68,
17,
68,
21,
2934,
15,
198,
2,
2343,
243,
254,
28670,
5705,
68,
22,
68,
23,
3388,
12,
15,
69,
19881,
12,
2231,
3829,
12,
64,
23,
2934,
12,
25270,
2816,
5332,
2791,
5333,
69,
198,
2,
2343,
243,
254,
28670,
66,
20,
558,
22,
344,
12,
12993,
64,
18,
12,
19,
66,
1314,
12,
65,
535,
16,
12,
23,
65,
2791,
324,
16,
66,
1433,
68,
24,
198,
2,
2343,
243,
254,
28670,
66,
19,
67,
4310,
23148,
12,
3070,
65,
16,
12,
19,
344,
19,
12,
64,
2920,
64,
12,
3388,
2931,
2231,
2682,
4524,
2624,
198,
2,
2343,
243,
254,
28670,
21,
330,
69,
19881,
24,
65,
12,
69,
23,
330,
12,
2598,
5999,
12,
23,
66,
6052,
12,
21738,
1238,
67,
15,
67,
24,
69,
15,
68,
198,
2,
2343,
243,
254,
28670,
18,
65,
3132,
69,
39322,
12,
22521,
17,
12,
19,
64,
22,
64,
12,
4531,
17896,
12,
23815,
65,
2548,
69,
17,
64,
4869,
68,
198,
2,
2343,
243,
254,
28670,
65,
21,
66,
20,
19420,
64,
12,
18,
67,
3901,
12,
22148,
24,
12,
397,
24,
65,
12,
67,
44218,
29119,
68,
15,
35809,
198,
2,
2343,
243,
254,
28670,
21,
66,
20,
34938,
15630,
12,
18,
67,
397,
12,
3559,
1558,
12,
2079,
66,
24,
12,
16,
11848,
2624,
65,
22883,
69,
15630,
198,
2,
2343,
243,
254,
28670,
18,
65,
16,
11848,
23451,
12,
2791,
69,
24,
12,
1821,
17457,
12,
64,
15,
64,
20,
12,
64,
18,
66,
19,
68,
16,
64,
23721,
1129,
198,
2,
2343,
243,
254,
28670,
65,
6659,
65,
1959,
2713,
12,
21,
38219,
12,
43489,
65,
12,
65,
16,
1350,
12,
66,
4051,
27728,
7568,
23,
65,
18,
69,
198,
2,
2343,
243,
253,
7280,
1765,
17,
67,
24,
69,
21,
64,
12,
23,
22842,
12,
42363,
64,
12,
64,
48597,
12,
65,
19,
69,
405,
1129,
67,
23,
28072,
198,
2,
2343,
243,
254,
28670,
22,
2682,
65,
3609,
1120,
12,
1065,
13331,
12,
2857,
1558,
12,
23,
27260,
12,
2860,
65,
4846,
18,
65,
23,
45758,
198,
2,
2343,
243,
254,
28670,
23,
2934,
22,
1433,
65,
24,
12,
6888,
3980,
12,
3682,
69,
20,
12,
64,
1765,
69,
12,
2670,
324,
24669,
69,
3510,
1485,
198,
2,
2343,
243,
254,
28670,
47984,
65,
891,
19881,
12,
64,
15982,
12,
19,
66,
4531,
12,
64,
19,
891,
12,
15,
1765,
2425,
2996,
1558,
66,
16,
68,
198,
2,
2343,
243,
254,
28670,
25838,
67,
1415,
3132,
12,
721,
2670,
12,
19,
67,
16,
68,
12,
6420,
16344,
12,
68,
36629,
891,
66,
16,
69,
20,
66,
18,
198,
2,
2343,
243,
254,
28670,
36434,
3270,
65,
16,
68,
12,
7012,
2624,
12,
2920,
67,
18,
12,
65,
15,
69,
16,
12,
20,
2414,
9945,
15,
2718,
7029,
66,
198,
2,
2343,
243,
254,
28670,
28676,
13381,
3104,
12,
3609,
24,
64,
12,
19,
397,
15,
12,
23,
69,
4089,
12,
5774,
69,
721,
1065,
66,
23,
3134,
64,
198,
2,
2343,
243,
254,
28670,
26429,
66,
16,
67,
1065,
12,
4309,
1558,
12,
32642,
68,
12,
65,
18,
64,
21,
12,
21,
69,
40839,
67,
1959,
65,
47576,
198,
2,
2343,
243,
254,
28670,
37452,
2682,
69,
405,
12,
2231,
67,
20,
12,
19,
12993,
24,
12,
5824,
69,
20,
12,
4304,
35175,
67,
19,
68,
4051,
2623,
198,
2,
2343,
243,
254,
28670,
3609,
43564,
64,
2075,
12,
66,
43690,
12,
38073,
64,
12,
65,
22995,
12,
64,
15,
1878,
721,
5892,
68,
3510,
69,
198,
2,
2343,
243,
254,
28670,
29279,
25600,
68,
12,
68,
5705,
65,
12,
19,
69,
2670,
12,
24,
344,
23,
12,
15,
68,
1238,
68,
3695,
64,
2919,
6052,
198,
2,
2343,
243,
254,
28670,
4531,
66,
17457,
20,
66,
15,
12,
15,
7568,
21,
12,
3559,
67,
18,
12,
17457,
20,
66,
12,
67,
18,
67,
2816,
67,
36657,
69,
2091,
198,
2,
2343,
243,
254,
28670,
69,
20,
3901,
65,
21536,
12,
18,
891,
23,
12,
7029,
18,
12,
65,
31128,
12,
15,
2996,
68,
20,
66,
3270,
2920,
324,
198,
2,
2343,
243,
254,
28670,
48057,
65,
11645,
65,
12,
15,
66,
16,
68,
12,
2780,
15630,
12,
330,
15,
65,
12,
17,
1453,
22,
16072,
16,
68,
397,
17,
65,
198,
2,
2343,
243,
254,
28670,
21,
67,
1314,
66,
17,
64,
19,
12,
21,
67,
16,
68,
12,
19,
65,
4531,
12,
65,
24,
68,
23,
12,
721,
487,
22914,
66,
2857,
1270,
198,
2,
2343,
243,
254,
28670,
36879,
69,
22709,
67,
12,
18638,
65,
12,
2857,
330,
12,
3829,
7568,
12,
66,
3270,
3720,
14877,
24038,
66,
198,
2,
2343,
243,
254,
28670,
34135,
3865,
67,
4869,
12,
21,
22172,
12,
2598,
65,
18,
12,
4531,
67,
16,
12,
22,
18213,
22,
69,
16,
65,
21,
1860,
21855,
198,
2,
2343,
243,
254,
28670,
64,
4349,
10210,
16,
17896,
12,
1120,
11848,
12,
2598,
2598,
12,
64,
15,
69,
22,
12,
66,
15,
68,
19,
23539,
65,
1065,
3553,
198,
2,
2343,
243,
254,
28670,
18182,
47101,
67,
20,
12,
66,
44550,
12,
2598,
4304,
12,
23,
69,
3104,
12,
69,
3553,
5333,
64,
2231,
2780,
4309,
198,
2,
2343,
243,
254,
28670,
24,
64,
21,
69,
43690,
68,
12,
68,
1120,
68,
12,
19,
40035,
12,
64,
16,
67,
16,
12,
18,
67,
17,
67,
3559,
16072,
5237,
16072,
198,
2,
2343,
243,
254,
28670,
23,
64,
21,
68,
535,
69,
15,
12,
5774,
1821,
12,
19,
67,
3388,
12,
65,
4846,
65,
12,
1065,
2780,
41948,
67,
19,
66,
19,
67,
198,
2,
2343,
243,
253,
7280,
2919,
64,
2931,
4761,
64,
12,
2816,
487,
12,
3682,
66,
24,
12,
23,
2548,
65,
12,
16,
64,
33300,
8635,
24,
64,
3510,
198,
2,
2343,
243,
254,
28670,
17,
67,
22,
6814,
2816,
68,
12,
1983,
1157,
12,
2598,
64,
19,
12,
65,
23,
65,
24,
12,
43444,
5066,
2481,
64,
19,
66,
2780,
198,
2,
2343,
243,
254,
28670,
4531,
13331,
18,
20219,
12,
16,
67,
940,
12,
19,
1860,
18,
12,
3829,
65,
16,
12,
67,
24,
66,
15,
69,
37680,
2231,
66,
21,
198,
2,
2343,
243,
254,
28670,
68,
24,
43552,
330,
12,
44617,
69,
12,
36260,
67,
12,
397,
15,
66,
12,
64,
1795,
2414,
69,
3553,
2623,
3695,
198,
2,
2343,
243,
254,
28670,
19,
10210,
41544,
65,
16,
12,
891,
1765,
12,
31794,
65,
12,
64,
36629,
12,
3324,
67,
15,
1453,
24,
67,
17,
65,
16,
65,
198,
2,
2343,
243,
254,
28670,
1350,
22,
67,
18,
19881,
20,
12,
67,
43950,
12,
1821,
68,
22,
12,
23,
25948,
12,
40090,
2091,
4051,
34770,
69,
23,
198,
2,
2343,
243,
254,
28670,
15,
19881,
2920,
3070,
66,
12,
2998,
19,
67,
12,
3901,
65,
23,
12,
64,
19,
68,
23,
12,
3365,
2682,
344,
23,
36445,
67,
20,
198,
2,
2343,
243,
254,
28670,
23,
64,
22,
64,
1731,
68,
18,
12,
2548,
4846,
12,
21844,
17,
12,
23,
68,
1120,
12,
37804,
64,
2154,
721,
18,
64,
2598,
198,
2,
2343,
243,
254,
28670,
19,
66,
17457,
1795,
4051,
12,
67,
16,
66,
22,
12,
19,
19924,
12,
3609,
5333,
12,
891,
2931,
344,
21,
7012,
24,
68,
22,
198,
2,
2343,
243,
254,
28670,
65,
5705,
1959,
1899,
69,
12,
891,
5333,
12,
19,
64,
2154,
12,
23,
64,
1558,
12,
20,
26598,
721,
18,
68,
20,
276,
18,
198,
2,
2343,
243,
254,
28670,
1731,
26276,
68,
2682,
12,
16072,
16,
67,
12,
19,
1453,
17,
12,
5774,
65,
21,
12,
1878,
65,
21,
64,
21495,
1314,
4846,
198,
2,
2343,
243,
254,
28670,
15,
69,
22,
68,
2623,
21101,
12,
66,
19,
64,
22,
12,
3559,
65,
20,
12,
24,
2078,
69,
12,
67,
26780,
65,
6469,
67,
28727,
65,
198,
2,
2343,
243,
254,
28670,
18,
65,
7568,
21,
2481,
68,
12,
38819,
65,
12,
3682,
65,
23,
12,
5332,
69,
23,
12,
20,
21142,
2075,
1433,
3510,
67,
18,
198,
2,
2343,
243,
254,
28670,
3682,
64,
16,
69,
535,
67,
12,
68,
46519,
12,
3682,
21855,
12,
6420,
2934,
12,
1507,
66,
23,
65,
2091,
67,
35378,
67,
198
] | 1.828275 | 12,520 |
# MGH problem 35 - Chebyquad function
#
# Source:
# J. J. Moré, B. S. Garbow and K. E. Hillstrom
# Testing Unconstrained Optimization Software
# ACM Transactions on Mathematical Software, 7(1):17-41, 1981
#
# A. Montoison, Montreal, 05/2018.
export mgh35
"Chebyquad function"
function mgh35(m :: Int = 10, n :: Int = 10)
if m < n
warn(": number of function must be ≥ number of variables. Adjusting to m = n")
m = n
end
nls = Model()
x0 = collect(1:n)/(n+1)
@variable(nls, x[i=1:n], start = x0[i])
function Tsim(x, n)
if n == 0
return 1
elseif n == 1
return x
else
return 2*x*Tsim(x,n-1) - Tsim(x,n-2)
end
end
Ts = Vector{Function}(undef, n)
Tnames = Vector{Symbol}(undef, n)
for i = 1:n
Ts[i] = x -> Tsim(2*x-1, i)
Tnames[i] = gensym()
JuMP.register(nls, Tnames[i], 1, Ts[i], autodiff=true)
end
I = [i%2 == 0 ? -1/(i^2-1) : 0 for i = 1:n]
@NLexpression(nls, F[i=1:n], sum($(Tnames[i])(x[j]) for j = 1:n)/n - I[i])
return MathProgNLSModel(nls, F, name="mgh35")
end
| [
2,
337,
17511,
1917,
3439,
532,
2580,
1525,
47003,
2163,
198,
2,
198,
2,
220,
220,
8090,
25,
198,
2,
220,
220,
449,
13,
449,
13,
3461,
2634,
11,
347,
13,
311,
13,
7164,
8176,
290,
509,
13,
412,
13,
3327,
20282,
198,
2,
220,
220,
23983,
791,
1102,
2536,
1328,
30011,
1634,
10442,
198,
2,
220,
220,
7125,
44,
46192,
319,
30535,
605,
10442,
11,
767,
7,
16,
2599,
1558,
12,
3901,
11,
14745,
198,
2,
198,
2,
317,
13,
5575,
78,
1653,
11,
12871,
11,
8870,
14,
7908,
13,
198,
198,
39344,
285,
456,
2327,
198,
198,
1,
7376,
1525,
47003,
2163,
1,
198,
8818,
285,
456,
2327,
7,
76,
7904,
2558,
796,
838,
11,
299,
7904,
2558,
796,
838,
8,
198,
220,
611,
285,
1279,
299,
198,
220,
220,
220,
9828,
7,
1298,
1271,
286,
2163,
1276,
307,
26870,
1271,
286,
9633,
13,
20292,
278,
284,
285,
796,
299,
4943,
198,
220,
220,
220,
285,
796,
299,
198,
220,
886,
628,
220,
299,
7278,
220,
796,
9104,
3419,
198,
220,
2124,
15,
220,
220,
796,
2824,
7,
16,
25,
77,
20679,
7,
77,
10,
16,
8,
198,
220,
2488,
45286,
7,
77,
7278,
11,
2124,
58,
72,
28,
16,
25,
77,
4357,
923,
796,
2124,
15,
58,
72,
12962,
628,
220,
2163,
13146,
320,
7,
87,
11,
299,
8,
198,
220,
220,
220,
611,
299,
6624,
657,
198,
220,
220,
220,
220,
220,
1441,
352,
198,
220,
220,
220,
2073,
361,
299,
6624,
352,
198,
220,
220,
220,
220,
220,
1441,
2124,
198,
220,
220,
220,
2073,
198,
220,
220,
220,
220,
220,
1441,
362,
9,
87,
9,
51,
14323,
7,
87,
11,
77,
12,
16,
8,
532,
13146,
320,
7,
87,
11,
77,
12,
17,
8,
198,
220,
220,
220,
886,
198,
220,
886,
628,
220,
13146,
796,
20650,
90,
22203,
92,
7,
917,
891,
11,
299,
8,
198,
220,
309,
14933,
796,
20650,
90,
13940,
23650,
92,
7,
917,
891,
11,
299,
8,
198,
220,
329,
1312,
796,
352,
25,
77,
198,
220,
220,
220,
13146,
58,
72,
60,
796,
2124,
4613,
13146,
320,
7,
17,
9,
87,
12,
16,
11,
1312,
8,
198,
220,
220,
220,
309,
14933,
58,
72,
60,
796,
308,
641,
4948,
3419,
198,
220,
220,
220,
12585,
7378,
13,
30238,
7,
77,
7278,
11,
309,
14933,
58,
72,
4357,
352,
11,
13146,
58,
72,
4357,
1960,
375,
733,
28,
7942,
8,
198,
220,
886,
628,
220,
314,
796,
685,
72,
4,
17,
6624,
657,
5633,
532,
16,
29006,
72,
61,
17,
12,
16,
8,
1058,
657,
329,
1312,
796,
352,
25,
77,
60,
198,
220,
2488,
32572,
38011,
7,
77,
7278,
11,
376,
58,
72,
28,
16,
25,
77,
4357,
2160,
16763,
7,
51,
14933,
58,
72,
60,
5769,
87,
58,
73,
12962,
329,
474,
796,
352,
25,
77,
20679,
77,
532,
314,
58,
72,
12962,
628,
220,
1441,
16320,
2964,
70,
45,
6561,
17633,
7,
77,
7278,
11,
376,
11,
1438,
2625,
76,
456,
2327,
4943,
198,
437,
198
] | 2.128 | 500 |