text
stringlengths 46
74.6k
|
---|
# Runtime
Runtime layer is used to execute smart contracts and other actions created by the users and preserve the state between the executions.
It can be described from three different angles: going step-by-step through various scenarios, describing the components
of the runtime, and describing the functions that the runtime performs.
## Scenarios
- Financial transaction -- we examine what happens when the runtime needs to process a simple financial transaction;
- Cross-contract call -- the scenario when the user calls a contract that in turn calls another contract.
## Components
The components of the runtime can be described through the crates:
- `near-vm-logic` -- describes the interface that smart contract uses to interact with the blockchain.
Encapsulates the behavior of the blockchain visible to the smart contract, e.g. fee rules, storage access rules, promise rules;
- `near-vm-runner` crate -- a wrapper around Wasmer that does the actual execution of the smart contract code. It exposes the
interface provided by `near-vm-logic` to the smart contract;
- `runtime` crate -- encapsulates the logic of how transactions and receipts should be handled. If it encounters
a smart contract call within a transaction or a receipt it calls `near-vm-runner`, for all other actions, like account
creation, it processes them in-place.
The utility crates are:
- `near-runtime-fees` -- a convenience crate that encapsulates configuration of fees. We might get rid ot it later;
- `near-vm-errors` -- contains the hierarchy of errors that can be occurred during transaction or receipt processing;
- `near-vm-runner-standalone` -- a runnable tool that allows running the runtime without the blockchain, e.g. for
integration testing of L2 projects;
- `runtime-params-estimator` -- benchmarks the runtime and generates the config with the fees.
Separately, from the components we describe [the Bindings Specification](Components/BindingsSpec/BindingsSpec.md) which is an
important part of the runtime that specifies the functions that the smart contract can call from its host -- the runtime.
The specification is defined in `near-vm-logic`, but it is exposed to the smart contract in `near-vm-runner`.
## Functions
- Receipt consumption and production
- Fees
- Virtual machine
- Verification
|
```js
import { Wallet } from './near-wallet';
const TOKEN_CONTRACT_ADDRESS = "token.v2.ref-finance.near";
const wallet = new Wallet({ createAccessKeyFor: TOKEN_CONTRACT_ADDRESS });
await wallet.callMethod({
method: 'ft_transfer_call',
args: {
receiver_id: "v2.ref-finance.near",
amount: "100000000000000000",
msg: "",
},
contractId: TOKEN_CONTRACT_ADDRESS,
gas: 300000000000000,
deposit: 1
});
```
<details>
<summary>Example response</summary>
<p>
```json
'100000000000000000'
```
</p>
</details>
_The `Wallet` object comes from our [quickstart template](https://github.com/near-examples/hello-near-examples/blob/main/frontend/near-wallet.js)_ |
---
id: events
title: Events
---
import {Github} from "@site/src/components/codetabs"
In this tutorial, you'll learn about the [events standard](https://nomicon.io/Standards/Tokens/NonFungibleToken/Event) and how to implement it in your smart contract.
---
## Understanding the use case {#understanding-the-use-case}
Have you ever wondered how the wallet knows which NFTs you own and how it can display them in the [collectibles tab](https://testnet.mynearwallet.com//?tab=collectibles)? Originally, an [indexer](/tools/indexer-for-explorer) was used and it listened for any functions starting with `nft_` on your account. These contracts were then flagged on your account as likely NFT contracts.
When you navigated to your collectibles tab, the wallet would then query all those contracts for the list of NFTs you owned using the `nft_tokens_for_owner` function you saw in the [enumeration tutorial](/tutorials/nfts/enumeration).
<hr class="subsection" />
### The problem {#the-problem}
This method of flagging contracts was not reliable as each NFT-driven application might have its own way of minting or transferring NFTs. In addition, it's common for apps to transfer or mint many tokens at a time using batch functions.
<hr class="subsection" />
### The solution {#the-solution}
A standard was introduced so that smart contracts could emit an event anytime NFTs were transferred, minted, or burnt. This event was in the form of a log. No matter how a contract implemented the functionality, an indexer could now listen for those standardized logs.
As per the standard, you need to implement a logging functionality that gets fired when NFTs are transferred or minted. In this case, the contract doesn't support burning so you don't need to worry about that for now.
It's important to note the standard dictates that the log should begin with `"EVENT_JSON:"`. The structure of your log should, however, always contain the 3 following things:
- **standard**: the current name of the standard (e.g. nep171)
- **version**: the version of the standard you're using (e.g. 1.0.0)
- **event**: a list of events you're emitting.
The event interface differs based on whether you're recording transfers or mints. The interface for both events is outlined below.
**Transfer events**:
- *Optional* - **authorized_id**: the account approved to transfer on behalf of the owner.
- **old_owner_id**: the old owner of the NFT.
- **new_owner_id**: the new owner that the NFT is being transferred to.
- **token_ids**: a list of NFTs being transferred.
- *Optional* - **memo**: an optional message to include with the event.
**Minting events**:
- **owner_id**: the owner that the NFT is being minted to.
- **token_ids**: a list of NFTs being transferred.
- *Optional* - **memo**: an optional message to include with the event.
<hr class="subsection" />
### Examples {#examples}
In order to solidify your understanding of the standard, let's walk through three scenarios and see what the logs should look like.
#### Scenario A - simple mint
In this scenario, Benji wants to mint an NFT to Mike with a token ID `"team-token"` and he doesn't include a message. The log should look as follows.
```rust
EVENT_JSON:{
"standard": "nep171",
"version": "1.0.0",
"event": "nft_mint",
"data": [
{"owner_id": "mike.testnet", "token_ids": ["team-token"]}
]
}
```
#### Scenario B - batch mint
In this scenario, Benji wants to perform a batch mint. He will mint an NFT to Mike, Damian, Josh, and Dorian. Dorian, however, will get two NFTs. Each token ID will be `"team-token"` followed by an incrementing number. The log is as follows.
```rust
EVENT_JSON:{
"standard": "nep171",
"version": "1.0.0",
"event": "nft_mint",
"data": [
{"owner_id": "mike.testnet", "token_ids": ["team-token0"]},
{"owner_id": "damian.testnet", "token_ids": ["team-token1"]},
{"owner_id": "josh.testnet", "token_ids": ["team-token2"]}
{"owner_id": "dorian.testnet", "token_ids": ["team-token3", "team-token4"]},
]
}
```
#### Scenario C - transfer NFTs
In this scenario, Mike is transferring both his team tokens to Josh. The log should look as follows.
```rust
EVENT_JSON:{
"standard": "nep171",
"version": "1.0.0",
"event": "nft_transfer",
"data": [
{"old_owner_id": "mike.testnet", "new_owner_id": "josh.testnet", "token_ids": ["team-token", "team-token0"], "memo": "Go Team!"}
]
}
```
---
## Modifications to the contract {#modifications-to-the-contract}
At this point, you should have a good understanding of what the end goal should be so let's get to work! Open the repository and create a new file in the `nft-contract-basic/src` directory called `events.rs`. This is where your log structs will live.
If you wish to see the finished code of the events implementation, that can be found on the `nft-contract-events` folder.
### Creating the events file {#events-rs}
Copy the following into your file. This will outline the structs for your `EventLog`, `NftMintLog`, and `NftTransferLog`. In addition, we've added a way for `EVENT_JSON:` to be prefixed whenever you log the `EventLog`.
<Github language="rust" start="1" end="79" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-events/src/events.rs" />
This requires the `serde_json` package which you can easily add to your `nft-contract-skeleton/Cargo.toml` file:
<Github language="rust" start="10" end="12" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-events/Cargo.toml" />
<hr class="subsection" />
### Adding modules and constants {#lib-rs}
Now that you've created a new file, you need to add the module to the `lib.rs` file. In addition, you can define two constants for the standard and version that will be used across our contract.
<Github language="rust" start="10" end="30" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-events/src/lib.rs" />
<hr class="subsection" />
### Logging minted tokens {#logging-minted-tokens}
Now that all the tools are set in place, you can now implement the actual logging functionality. Since the contract will only be minting tokens in one place, open the `nft-contract-basic/src/mint.rs` file and navigate to the bottom of the file. This is where you'll construct the log for minting. Anytime someone successfully mints an NFT, it will now correctly emit a log.
<Github language="rust" start="5" end="58" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-events/src/mint.rs" />
<hr class="subsection" />
### Logging transfers {#logging-transfers}
Let's open the `nft-contract-basic/src/internal.rs` file and navigate to the `internal_transfer` function. This is the location where you'll build your transfer logs. Whenever an NFT is transferred, this function is called and so you'll correctly be logging the transfers.
<Github language="rust" start="96" end="159" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-events/src/internal.rs" />
This solution, unfortunately, has an edge case which will break things. If an NFT is transferred via the `nft_transfer_call` function, there's a chance that the transfer will be reverted if the `nft_on_transfer` function returns `true`. Taking a look at the logic for `nft_transfer_call`, you can see why this is a problem.
When `nft_transfer_call` is invoked, it will:
- Call `internal_transfer` to perform the actual transfer logic.
- Initiate a cross-contract call and invoke the `nft_on_transfer` function.
- Resolve the promise and perform logic in `nft_resolve_transfer`.
- This will either return true meaning the transfer went fine or it will revert the transfer and return false.
If you only place the log in the `internal_transfer` function, the log will be emitted and the indexer will think that the NFT was transferred. If the transfer is reverted during `nft_resolve_transfer`, however, that event should **also** be emitted. Anywhere that an NFT **could** be transferred, we should add logs. Replace the `nft_resolve_transfer` with the following code.
<Github language="rust" start="157" end="241" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-events/src/nft_core.rs" />
In addition, you need to add an `authorized_id` and `memo` to the parameters for `nft_resolve_transfer` as shown below.
:::tip
We will talk more about this [`authorized_id`](./5-approval.md) in the following chapter.
:::
<Github language="rust" start="43" end="60" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-events/src/nft_core.rs" />
The last step is to modify the `nft_transfer_call` logic to include these new parameters:
<Github language="rust" start="86" end="135" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-events/src/nft_core.rs" />
With that finished, you've successfully implemented the events standard and it's time to start testing.
---
## Deploying the contract {#redeploying-contract}
For the purpose of readability and ease of development, instead of redeploying the contract to the same account, let's create an account and deploy to that instead. You could have deployed to the same account as none of the changes you implemented in this tutorial would have caused errors.
### Deployment
Next, you'll deploy this contract to the network.
```bash
export EVENTS_NFT_CONTRACT_ID=<accountId>
near create-account $EVENTS_NFT_CONTRACT_ID --useFaucet
```
Using the cargo-near, deploy and initialize the contract as you did in the previous tutorials:
```bash
cargo near deploy $EVENTS_NFT_CONTRACT_ID with-init-call new_default_meta json-args '{"owner_id": "'$EVENTS_NFT_CONTRACT_ID'"}' prepaid-gas '100.0 Tgas' attached-deposit '0 NEAR' network-config testnet sign-with-keychain send
```
<hr class="subsection" />
### Minting {#minting}
Next, you'll need to mint a token. By running this command, you'll mint a token with a token ID `"events-token"` and the receiver will be your new account. In addition, you're passing in a map with two accounts that will get perpetual royalties whenever your token is sold.
```bash
near call $EVENTS_NFT_CONTRACT_ID nft_mint '{"token_id": "events-token", "metadata": {"title": "Events Token", "description": "testing out the new events extension of the standard", "media": "https://bafybeiftczwrtyr3k7a2k4vutd3amkwsmaqyhrdzlhvpt33dyjivufqusq.ipfs.dweb.link/goteam-gif.gif"}, "receiver_id": "'$EVENTS_NFT_CONTRACT_ID'"}' --accountId $EVENTS_NFT_CONTRACT_ID --amount 0.1
```
You can check to see if everything went through properly by looking at the output in your CLI:
```bash
Doing account.functionCall()
Receipts: F4oxNfv54cqwUwLUJ7h74H1iE66Y3H7QDfZMmGENwSxd, BJxKNFRuLDdbhbGeLA3UBSbL8UicU7oqHsWGink5WX7S
Log [events.goteam.examples.testnet]: EVENT_JSON:{"standard":"nep171","version":"1.0.0","event":"nft_mint","data":[{"owner_id":"events.goteam.examples.testnet","token_ids":["events-token"]}]}
Transaction Id 4Wy2KQVTuAWQHw5jXcRAbrz7bNyZBoiPEvLcGougciyk
To see the transaction in the transaction explorer, please open this url in your browser
https://testnet.nearblocks.io/txns/4Wy2KQVTuAWQHw5jXcRAbrz7bNyZBoiPEvLcGougciyk
''
```
You can see that the event was properly logged!
<hr class="subsection" />
### Transferring {#transferring}
You can now test if your transfer log works as expected by sending `benjiman.testnet` your NFT.
```bash
near call $EVENTS_NFT_CONTRACT_ID nft_transfer '{"receiver_id": "benjiman.testnet", "token_id": "events-token", "memo": "Go Team :)", "approval_id": 0}' --accountId $EVENTS_NFT_CONTRACT_ID --depositYocto 1
```
This should return an output similar to the following:
```bash
Doing account.functionCall()
Receipts: EoqBxrpv9Dgb8KqK4FdeREawVVLWepEUR15KPNuZ4fGD, HZ4xQpbgc8EfU3PiV72LvfXb2f3dVC1n9aVTbQds9zfR
Log [events.goteam.examples.testnet]: Memo: Go Team :)
Log [events.goteam.examples.testnet]: EVENT_JSON:{"standard":"nep171","version":"1.0.0","event":"nft_transfer","data":[{"authorized_id":"events.goteam.examples.testnet","old_owner_id":"events.goteam.examples.testnet","new_owner_id":"benjiman.testnet","token_ids":["events-token"],"memo":"Go Team :)"}]}
Transaction Id 4S1VrepKzA6HxvPj3cK12vaT7Dt4vxJRWESA1ym1xdvH
To see the transaction in the transaction explorer, please open this url in your browser
https://testnet.nearblocks.io/txns/4S1VrepKzA6HxvPj3cK12vaT7Dt4vxJRWESA1ym1xdvH
''
```
Hurray! At this point, your NFT contract is fully complete and the events standard has been implemented.
---
## Conclusion
Today you went through the [events standard](https://nomicon.io/Standards/Tokens/NonFungibleToken/Event) and implemented the necessary logic in your smart contract. You created events for [minting](#logging-minted-tokens) and [transferring](#logging-transfers) NFTs. You then deployed and [tested](#initialization-and-minting) your changes by minting and transferring NFTs.
In the next tutorial, you'll look at the basics of a marketplace contract and how it was built.
:::note Versioning for this article
At the time of this writing, this example works with the following versions:
- near-cli: `4.0.13`
- cargo-near `0.6.1`
- NFT standard: [NEP171](https://nomicon.io/Standards/Tokens/NonFungibleToken/Core), version `1.1.0`
- Events standard: [NEP297 extension](https://nomicon.io/Standards/Tokens/NonFungibleToken/Event), version `1.1.0`
:::
|
NEAR Lunch & Learn Ep. 03: Light clients in Proof-of-Stake systems
COMMUNITY
November 14, 2019
In this video we discuss the challenges of implementing light clients in Proof-of-Stake systems that either do not use BFT consensus at all, or use some sort of BFT finality gadget, but allow a large sequence of blocks to be created without any of them being finalized.
We start by covering light clients in Proof of Work systems. In a Proof of Work system for as long as the client can verify proofs of work in the headers, it is extremely complex for an adversary to convince a light client that a particular chain is canonical without executing an Eclipse attack, or carrying out a several hours long 51% attack.
We then cover light clients in BFT systems, in which each block is finalized. In such a system the light client can just confirm that each block has the signature of a super majority of validators, and confirm locally that the validator set transitions were executed properly. Unless the adversary at some point controlled a super majority of validators, or executed a long-range attack, they cannot trick a light client into believing that an adversarial chain is canonical.
Finally, we cover a major challenge of designing a light client in a proof of stake system that doesn’t use BFT finality, or allows epochs without finalized blocks. We argue that to support the clients a Proof-of-Stake system either needs to require each epoch to have at least one block finalized, or to use some scarce resource (such as work or space) in addition to Proof-of-Stake.
|
Happy Hacking | November 15th, 2019
COMMUNITY
November 15, 2019
We have just opened the registration for the next week of Stake Wars. If you are interested in participating, please join us on Discord; you will find more information in the validator channel. So far we have received lots of feedback that allowed us to improve the testnet, fix bugs, and make the program more accessible for everyone. If you are thinking of joining, please have a try, the Near team is here to support.
The third episode of our “Lunch & Learn” series is out, in which Alex discusses the challenges of implementing light clients in Proof-of-Stake systems. In “Lunch & Learn” Illia and Alex share the design, assumptions, and implementation of NEAR Protocol.
Lastly, we are increasing our efforts to gather community and developer feedback. If you are in the Bay Area, please pass by our office for one of our “Blockchain Hack Nights”. The first one will happen next week – Sign-up here!
For everyone who cannot make it in person, we would still love to hear from you. Please fill out the following form. Someone from the team will get in touch.
“Lunch & Learn” third episode
COMMUNITY UPDATE
Our goal is to build a self-sufficient community. To get there, we are in the process of changing the structure of the ambassador program to give local hubs more autonomy and to operate independently from Near.
The Future of Blockchain Hackathon has started! We currently have 30 teams signed-up to build on NEAR. Some teams are looking for additional team members; if you are interested, have a look at the overview and join the fob-hackathon channel in our Discord.
UPCOMING EVENTS
We are launching “Blockchain Hack Night”; a bi-weekly meetup for developers and blockchain enthusiasts in the Bay Area. Besides talks on dApp and blockchain development, we will help you get started building your own dApp on NEAR. The first one will happen next week – Sign-up now!
Join us here!
WRITING AND CONTENT
Have a look at Gavin’s article, summarising the AMA between Staking Hub and Illia. Take me to the article.
To learn more about our approach to sharding, have a look at Alex’ talk at the second annual Scalar Capital summit.
We have more ambassadors from Europe getting involved. A big shout-out to Markus, who has started a German Medium site; articles include a German translation of “The Beginner’s Guide to the NEAR Blockchain”.
“Early morning in @NEARProtocol HQ” – Tweet
ENGINEERING UPDATES
156 PRs across 23 repos by 27 authors. Featured repos:nearcore,nearlib,near-shell,near-wallet,near-bindgen, assemblyscript-json, nomicon, near-ops, near-contract-helper, borsh, stakewars and near-explorer;
Release v0.4.5 of nearcore
Merklization of execution outcomes in nearcore
Reduce wait for validators who are offline, remove mem leak in nearcore
Finalizing the Finality Gadget in NEARCore — the finality gadget described in https://near.ai/post is now fully implemented, integrated and tested. Under normal circumstances whenever a block at height `k` is published, the block at height `k-2` is irreversible.
The final PR in implementing proper state sync in nearcore.
near-shell 0.14.1 published
Updated transactions ordering doc, add an example in nomicon
Fixed server-side rendering of node pages in near-explorer
A three-part PR (1, 2 and 3) improving transaction pool and implementing delaying receipts to prevent spamming shards in nearcore
Major overhaul of networking code in nearcore
Implement runtime fee per touched trie node in nearcore
NEP outlining the details of the Proposed implementation of the randomness beacon
Node & Staking and visual changes in near-wallet
HOW YOU CAN GET INVOLVED
Join us: there are new jobs we’re hiring across the board!
If you want to work with one of the most talented teams in the world right now to solve incredibly hard problems, check out our careers page for openings. And tell your friends!
Learn more about NEAR in The Beginner’s Guide to NEAR Protocol. Stay up to date with what we’re building by following us on Twitter for updates, joining the conversation on Discord and subscribing to our newsletter to receive updates right to your inbox.
https://upscri.be/633436/ |
---
NEP: 245
Title: Multi Token Standard
Author: Zane Starr <zane@ships.gold>, @riqi, @jriemann, @marcos.sun
DiscussionsTo: https://github.com/near/NEPs/discussions/246
Status: Review
Type: Standards Track
Category: Contract
Created: 03-Mar-2022
Requires: 297
---
## Summary
A standard interface for a multi token standard that supports fungible, semi-fungible,non-fungible, and tokens of any type, allowing for ownership, transfer, and batch transfer of tokens regardless of specific type.
## Motivation
In the three years since [ERC-1155] was ratified by the Ethereum Community, Multi Token based contracts have proven themselves valuable assets. Many blockchain projects emulate this standard for representing multiple token assets classes in a single contract. The ability to reduce transaction overhead for marketplaces, video games, DAOs, and exchanges is appealing to the blockchain ecosystem and simplifies transactions for developers.
Having a single contract represent NFTs, FTs, and tokens that sit inbetween greatly improves efficiency. The standard also introduced the ability to make batch requests with multiple asset classes reducing complexity. This standard allows operations that currently require _many_ transactions to be completed in a single transaction that can transfer not only NFTs and FTs, but any tokens that are a part of same token contract.
With this standard, we have sought to take advantage of the ability of the NEAR blockchain to scale. Its sharded runtime, and [storage staking] model that decouples [gas] fees from storage demand, enables ultra low transaction fees and greater on chain storage (see [Metadata][MT Metadata] extension).
With the aforementioned, it is noteworthy to mention that like the [NFT] standard the Multi Token standard, implements `mt_transfer_call`,
which allows, a user to attach many tokens to a call to a separate contract. Additionally, this standard includes an optional [Approval Management] extension. The extension allows marketplaces to trade on behalf of a user, providing additional flexibility for dApps.
Prior art:
- [ERC-721]
- [ERC-1155]
- [NEAR Fungible Token Standard][FT Core], which first pioneered the "transfer and call" technique
- [NEAR Non-Fungible Token Standard][NFT Core]
## Rationale and alternatives
Why have another standard, aren't fungible and non-fungible tokens enough? The current fungible token and non-fungible token standards, do not provide support for representing many FT tokens in a single contract, as well as the flexibility to define different token types with different behavior in a single contract. This is something that makes it difficult to be interoperable with other major blockchain networks, that implement standards that allow for representation of many different FT tokens in a single contract such as Ethereum.
The standard here introduces a few concepts that evolve the original [ERC-1155] standard to have more utility, while maintaining the original flexibility of the standard. So keeping that in mind, we are defining this as a new token type. It combines two main features of FT and NFT. It allows us to represent many token types in a single contract, and it's possible to store the amount for each token.
The decision to not use FT and NFT as explicit token types was taken to allow the community to define their own standards and meanings through metadata. As standards evolve on other networks, this specification allows the standard to be able to represent tokens across networks accurately, without necessarily restricting the behavior to any preset definition.
The issues with this in general is a problem with defining what metadata means and how is that interpreted. We have chosen to follow the pattern that is currently in use on Ethereum in the [ERC-1155] standard. That pattern relies on people to make extensions or to make signals as to how they want the metadata to be represented for their use case.
One of the areas that has broad sweeping implications from the [ERC-1155] standard is the lack of direct access to metadata. With Near's sharding we are able to have a [Metadata Extension][MT Metadata] for the standard that exists on chain. So developers and users are not required to use an indexer to understand, how to interact or interpret tokens, via token identifiers that they receive.
Another extension that we made was to provide an explicit ability for developers and users to group or link together series of NFTs/FTs or any combination of tokens. This provides additional flexibility that the [ERC-1155] standard only has loose guidelines on. This was chosen to make it easy for consumers to understand the relationship between tokens within the contract.
To recap, we choose to create this standard, to improve interoperability, developer ease of use, and to extend token representability beyond what was available directly in the FT or NFT standards. We believe this to be another tool in the developer's toolkit. It makes it possible to represent many types of tokens and to enable exchanges of many tokens within a single `transaction`.
## Specification
**NOTES**:
- All amounts, balances and allowance are limited by `U128` (max value `2**128 - 1`).
- Token standard uses JSON for serialization of arguments and results.
- Amounts in arguments and results are serialized as Base-10 strings, e.g. `"100"`. This is done to avoid JSON limitation of max integer value of `2**53`.
- The contract must track the change in storage when adding to and removing from collections. This is not included in this core multi token standard but instead in the [Storage Standard][Storage Management].
- To prevent the deployed contract from being modified or deleted, it should not have any access keys on its account.
### MT Interface
```ts
// The base structure that will be returned for a token. If contract is using
// extensions such as Approval Management, Enumeration, Metadata, or other
// attributes may be included in this structure.
type Token = {
token_id: string,
owner_id: string | null
}
/******************/
/* CHANGE METHODS */
/******************/
// Simple transfer. Transfer a given `token_id` from current owner to
// `receiver_id`.
//
// Requirements
// * Caller of the method must attach a deposit of 1 yoctoⓃ for security purposes
// * Caller must have greater than or equal to the `amount` being requested
// * Contract MUST panic if called by someone other than token owner or,
// if using Approval Management, one of the approved accounts
// * `approval_id` is for use with Approval Management extension, see
// that document for full explanation.
// * If using Approval Management, contract MUST nullify approved accounts on
// successful transfer.
//
// Arguments:
// * `receiver_id`: the valid NEAR account receiving the token
// * `token_id`: the token to transfer
// * `amount`: the number of tokens to transfer, wrapped in quotes and treated
// like a string, although the number will be stored as an unsigned integer
// with 128 bits.
// * `approval` (optional): is a tuple of [`owner_id`,`approval_id`].
// `owner_id` is the valid Near account that owns the tokens.
// `approval_id` is the expected approval ID. A number smaller than
// 2^53, and therefore representable as JSON. See Approval Management
// standard for full explanation.
// * `memo` (optional): for use cases that may benefit from indexing or
// providing information for a transfer
function mt_transfer(
receiver_id: string,
token_id: string,
amount: string,
approval: [owner_id: string, approval_id: number]|null,
memo: string|null,
) {}
// Simple batch transfer. Transfer a given `token_ids` from current owner to
// `receiver_id`.
//
// Requirements
// * Caller of the method must attach a deposit of 1 yoctoⓃ for security purposes
// * Caller must have greater than or equal to the `amounts` being requested for the given `token_ids`
// * Contract MUST panic if called by someone other than token owner or,
// if using Approval Management, one of the approved accounts
// * `approval_id` is for use with Approval Management extension, see
// that document for full explanation.
// * If using Approval Management, contract MUST nullify approved accounts on
// successful transfer.
// * Contract MUST panic if called with the length of `token_ids` not equal to `amounts` is not equal
// * Contract MUST panic if `approval_ids` is not `null` and does not equal the length of `token_ids`
//
// Arguments:
// * `receiver_id`: the valid NEAR account receiving the token
// * `token_ids`: the tokens to transfer
// * `amounts`: the number of tokens to transfer, wrapped in quotes and treated
// like an array of strings, although the numbers will be stored as an array of unsigned integer
// with 128 bits.
// * `approvals` (optional): is an array of expected `approval` per `token_ids`.
// If a `token_id` does not have a corresponding `approval` then the entry in the array
// must be marked null.
// `approval` is a tuple of [`owner_id`,`approval_id`].
// `owner_id` is the valid Near account that owns the tokens.
// `approval_id` is the expected approval ID. A number smaller than
// 2^53, and therefore representable as JSON. See Approval Management
// standard for full explanation.
// * `memo` (optional): for use cases that may benefit from indexing or
// providing information for a transfer
function mt_batch_transfer(
receiver_id: string,
token_ids: string[],
amounts: string[],
approvals: ([owner_id: string, approval_id: number]| null)[]| null,
memo: string|null,
) {}
// Transfer token and call a method on a receiver contract. A successful
// workflow will end in a success execution outcome to the callback on the MT
// contract at the method `mt_resolve_transfer`.
//
// You can think of this as being similar to attaching native NEAR tokens to a
// function call. It allows you to attach any Multi Token, token in a call to a
// receiver contract.
//
// Requirements:
// * Caller of the method must attach a deposit of 1 yoctoⓃ for security
// purposes
// * Caller must have greater than or equal to the `amount` being requested
// * Contract MUST panic if called by someone other than token owner or,
// if using Approval Management, one of the approved accounts
// * The receiving contract must implement `mt_on_transfer` according to the
// standard. If it does not, MT contract's `mt_resolve_transfer` MUST deal
// with the resulting failed cross-contract call and roll back the transfer.
// * Contract MUST implement the behavior described in `mt_resolve_transfer`
// * `approval_id` is for use with Approval Management extension, see
// that document for full explanation.
// * If using Approval Management, contract MUST nullify approved accounts on
// successful transfer.
//
// Arguments:
// * `receiver_id`: the valid NEAR account receiving the token.
// * `token_id`: the token to send.
// * `amount`: the number of tokens to transfer, wrapped in quotes and treated
// like a string, although the number will be stored as an unsigned integer
// with 128 bits.
// * `owner_id`: the valid NEAR account that owns the token
// * `approval` (optional): is a tuple of [`owner_id`,`approval_id`].
// `owner_id` is the valid Near account that owns the tokens.
// `approval_id` is the expected approval ID. A number smaller than
// 2^53, and therefore representable as JSON. See Approval Management
// * `memo` (optional): for use cases that may benefit from indexing or
// providing information for a transfer.
// * `msg`: specifies information needed by the receiving contract in
// order to properly handle the transfer. Can indicate both a function to
// call and the parameters to pass to that function.
function mt_transfer_call(
receiver_id: string,
token_id: string,
amount: string,
approval: [owner_id: string, approval_id: number]|null,
memo: string|null,
msg: string,
): Promise {}
// Transfer tokens and call a method on a receiver contract. A successful
// workflow will end in a success execution outcome to the callback on the MT
// contract at the method `mt_resolve_transfer`.
//
// You can think of this as being similar to attaching native NEAR tokens to a
// function call. It allows you to attach any Multi Token, token in a call to a
// receiver contract.
//
// Requirements:
// * Caller of the method must attach a deposit of 1 yoctoⓃ for security
// purposes
// * Caller must have greater than or equal to the `amount` being requested
// * Contract MUST panic if called by someone other than token owner or,
// if using Approval Management, one of the approved accounts
// * The receiving contract must implement `mt_on_transfer` according to the
// standard. If it does not, MT contract's `mt_resolve_transfer` MUST deal
// with the resulting failed cross-contract call and roll back the transfer.
// * Contract MUST implement the behavior described in `mt_resolve_transfer`
// * `approval_id` is for use with Approval Management extension, see
// that document for full explanation.
// * If using Approval Management, contract MUST nullify approved accounts on
// successful transfer.
// * Contract MUST panic if called with the length of `token_ids` not equal to `amounts` is not equal
// * Contract MUST panic if `approval_ids` is not `null` and does not equal the length of `token_ids`
//
// Arguments:
// * `receiver_id`: the valid NEAR account receiving the token.
// * `token_ids`: the tokens to transfer
// * `amounts`: the number of tokens to transfer, wrapped in quotes and treated
// like an array of string, although the numbers will be stored as an array of
// unsigned integer with 128 bits.
// * `approvals` (optional): is an array of expected `approval` per `token_ids`.
// If a `token_id` does not have a corresponding `approval` then the entry in the array
// must be marked null.
// `approval` is a tuple of [`owner_id`,`approval_id`].
// `owner_id` is the valid Near account that owns the tokens.
// `approval_id` is the expected approval ID. A number smaller than
// 2^53, and therefore representable as JSON. See Approval Management
// standard for full explanation.
// * `memo` (optional): for use cases that may benefit from indexing or
// providing information for a transfer.
// * `msg`: specifies information needed by the receiving contract in
// order to properly handle the transfer. Can indicate both a function to
// call and the parameters to pass to that function.
function mt_batch_transfer_call(
receiver_id: string,
token_ids: string[],
amounts: string[],
approvals: ([owner_id: string, approval_id: number]|null)[] | null,
memo: string|null,
msg: string,
): Promise {}
/****************/
/* VIEW METHODS */
/****************/
// Returns the tokens with the given `token_ids` or `null` if no such token.
function mt_token(token_ids: string[]) (Token | null)[]
// Returns the balance of an account for the given `token_id`.
// The balance though wrapped in quotes and treated like a string,
// the number will be stored as an unsigned integer with 128 bits.
// Arguments:
// * `account_id`: the NEAR account that owns the token.
// * `token_id`: the token to retrieve the balance from
function mt_balance_of(account_id: string, token_id: string): string
// Returns the balances of an account for the given `token_ids`.
// The balances though wrapped in quotes and treated like strings,
// the numbers will be stored as an unsigned integer with 128 bits.
// Arguments:
// * `account_id`: the NEAR account that owns the tokens.
// * `token_ids`: the tokens to retrieve the balance from
function mt_batch_balance_of(account_id: string, token_ids: string[]): string[]
// Returns the token supply with the given `token_id` or `null` if no such token exists.
// The supply though wrapped in quotes and treated like a string, the number will be stored
// as an unsigned integer with 128 bits.
function mt_supply(token_id: string): string | null
// Returns the token supplies with the given `token_ids`, a string value is returned or `null`
// if no such token exists. The supplies though wrapped in quotes and treated like strings,
// the numbers will be stored as an unsigned integer with 128 bits.
function mt_batch_supply(token_ids: string[]): (string | null)[]
```
The following behavior is required, but contract authors may name this function something other than the conventional `mt_resolve_transfer` used here.
```ts
// Finalize an `mt_transfer_call` or `mt_batch_transfer_call` chain of cross-contract calls. Generically
// referred to as `mt_transfer_call` as it applies to `mt_batch_transfer_call` as well.
//
// The `mt_transfer_call` process:
//
// 1. Sender calls `mt_transfer_call` on MT contract
// 2. MT contract transfers token from sender to receiver
// 3. MT contract calls `mt_on_transfer` on receiver contract
// 4+. [receiver contract may make other cross-contract calls]
// N. MT contract resolves promise chain with `mt_resolve_transfer`, and may
// transfer token back to sender
//
// Requirements:
// * Contract MUST forbid calls to this function by any account except self
// * If promise chain failed, contract MUST revert token transfer
// * If promise chain resolves with `true`, contract MUST return token to
// `sender_id`
//
// Arguments:
// * `sender_id`: the sender of `mt_transfer_call`
// * `receiver_id`: the `receiver_id` argument given to `mt_transfer_call`
// * `token_ids`: the `token_ids` argument given to `mt_transfer_call`
// * `amounts`: the `token_ids` argument given to `mt_transfer_call`
// * `approvals (optional)`: if using Approval Management, contract MUST provide
// set of original approvals in this argument, and restore the
// approved accounts in case of revert.
// `approvals` is an array of expected `approval_list` per `token_ids`.
// If a `token_id` does not have a corresponding `approvals_list` then the entry in the
// array must be marked null.
// `approvals_list` is an array of triplets of [`owner_id`,`approval_id`,`amount`].
// `owner_id` is the valid Near account that owns the tokens.
// `approval_id` is the expected approval ID. A number smaller than
// 2^53, and therefore representable as JSON. See Approval Management
// standard for full explanation.
// `amount`: the number of tokens to transfer, wrapped in quotes and treated
// like a string, although the number will be stored as an unsigned integer
// with 128 bits.
//
//
//
// Returns total amount spent by the `receiver_id`, corresponding to the `token_id`.
// The amounts returned, though wrapped in quotes and treated like strings,
// the numbers will be stored as an unsigned integer with 128 bits.
// Example: if sender_id calls `mt_transfer_call({ "amounts": ["100"], token_ids: ["55"], receiver_id: "games" })`,
// but `receiver_id` only uses 80, `mt_on_transfer` will resolve with `["20"]`, and `mt_resolve_transfer`
// will return `["80"]`.
function mt_resolve_transfer(
sender_id: string,
receiver_id: string,
token_ids: string[],
approvals: (null | [owner_id: string, approval_id: number, amount: string][]) []| null
):string[] {}
```
### Receiver Interface
Contracts which want to make use of `mt_transfer_call` and `mt_batch_transfer_call` must implement the following:
```ts
// Take some action after receiving a multi token
//
// Requirements:
// * Contract MUST restrict calls to this function to a set of whitelisted
// contracts
// * Contract MUST panic if `token_ids` length does not equals `amounts`
// length
// * Contract MUST panic if `previous_owner_ids` length does not equals `token_ids`
// length
//
// Arguments:
// * `sender_id`: the sender of `mt_transfer_call`
// * `previous_owner_ids`: the account that owned the tokens prior to it being
// transferred to this contract, which can differ from `sender_id` if using
// Approval Management extension
// * `token_ids`: the `token_ids` argument given to `mt_transfer_call`
// * `amounts`: the `token_ids` argument given to `mt_transfer_call`
// * `msg`: information necessary for this contract to know how to process the
// request. This may include method names and/or arguments.
//
// Returns the number of unused tokens in string form. For instance, if `amounts`
// is `["10"]` but only 9 are needed, it will return `["1"]`. The amounts returned,
// though wrapped in quotes and treated like strings, the numbers will be stored as
// an unsigned integer with 128 bits.
function mt_on_transfer(
sender_id: string,
previous_owner_ids: string[],
token_ids: string[],
amounts: string[],
msg: string,
): Promise<string[]>;
```
## Events
NEAR and third-party applications need to track
`mint`, `burn`, `transfer` events for all MT-driven apps consistently. This exension addresses that.
Note that applications, including NEAR Wallet, could require implementing additional methods to display tokens correctly such as [`mt_metadata`][MT Metadata] and [`mt_tokens_for_owner`][MT Enumeration].
### Events Interface
Multi Token Events MUST have `standard` set to `"nep245"`, standard version set to `"1.0.0"`, `event` value is one of `mt_mint`, `mt_burn`, `mt_transfer`, and `data` must be of one of the following relevant types: `MtMintLog[] | MtBurnLog[] | MtTransferLog[]`:
```ts
interface MtEventLogData {
EVENT_JSON: {
standard: "nep245",
version: "1.0.0",
event: MtEvent,
data: MtMintLog[] | MtBurnLog[] | MtTransferLog[]
}
}
```
```ts
// Minting event log. Emitted when a token is minted/created.
// Requirements
// * Contract MUST emit event when minting a token
// Fields
// * Contract token_ids and amounts MUST be the same length
// * `owner_id`: the account receiving the minted token
// * `token_ids`: the tokens minted
// * `amounts`: the number of tokens minted, wrapped in quotes and treated
// like a string, although the numbers will be stored as an unsigned integer
// array with 128 bits.
// * `memo`: optional message
interface MtMintLog {
owner_id: string,
token_ids: string[],
amounts: string[],
memo?: string
}
// Burning event log. Emitted when a token is burned.
// Requirements
// * Contract MUST emit event when minting a token
// Fields
// * Contract token_ids and amounts MUST be the same length
// * `owner_id`: the account whose token(s) are being burned
// * `authorized_id`: approved account_id to burn, if applicable
// * `token_ids`: the tokens being burned
// * `amounts`: the number of tokens burned, wrapped in quotes and treated
// like a string, although the numbers will be stored as an unsigned integer
// array with 128 bits.
// * `memo`: optional message
interface MtBurnLog {
owner_id: string,
authorized_id?: string,
token_ids: string[],
amounts: string[],
memo?: string
}
// Transfer event log. Emitted when a token is transferred.
// Requirements
// * Contract MUST emit event when transferring a token
// Fields
// * `authorized_id`: approved account_id to transfer
// * `old_owner_id`: the account sending the tokens "sender.near"
// * `new_owner_id`: the account receiving the tokens "receiver.near"
// * `token_ids`: the tokens to transfer
// * `amounts`: the number of tokens to transfer, wrapped in quotes and treated
// like a string, although the numbers will be stored as an unsigned integer
// array with 128 bits.
interface MtTransferLog {
authorized_id?: string,
old_owner_id: string,
new_owner_id: string,
token_ids: string[],
amounts: string[],
memo?: string
}
```
## Examples
Single owner minting (pretty-formatted for readability purposes):
```js
EVENT_JSON:{
"standard": "nep245",
"version": "1.0.0",
"event": "mt_mint",
"data": [
{"owner_id": "foundation.near", "token_ids": ["aurora", "proximitylabs_ft"], "amounts":["1", "100"]}
]
}
```
Different owners minting:
```js
EVENT_JSON:{
"standard": "nep245",
"version": "1.0.0",
"event": "mt_mint",
"data": [
{"owner_id": "foundation.near", "token_ids": ["aurora", "proximitylabs_ft"], "amounts":["1","100"]},
{"owner_id": "user1.near", "token_ids": ["meme"], "amounts": ["1"]}
]
}
```
Different events (separate log entries):
```js
EVENT_JSON:{
"standard": "nep245",
"version": "1.0.0",
"event": "mt_burn",
"data": [
{"owner_id": "foundation.near", "token_ids": ["aurora", "proximitylabs_ft"], "amounts": ["1","100"]},
]
}
```
Authorized id:
```js
EVENT_JSON:{
"standard": "nep245",
"version": "1.0.0",
"event": "mt_burn",
"data": [
{"owner_id": "foundation.near", "token_ids": ["aurora_alpha", "proximitylabs_ft"], "amounts": ["1","100"], "authorized_id": "thirdparty.near" },
]
}
```
```js
EVENT_JSON:{
"standard": "nep245",
"version": "1.0.0",
"event": "mt_transfer",
"data": [
{"old_owner_id": "user1.near", "new_owner_id": "user2.near", "token_ids": ["meme"], "amounts":["1"], "memo": "have fun!"}
]
}
EVENT_JSON:{
"standard": "nep245",
"version": "1.0.0",
"event": "mt_transfer",
"data": [
{"old_owner_id": "user2.near", "new_owner_id": "user3.near", "token_ids": ["meme"], "amounts":["1"], "authorized_id": "thirdparty.near", "memo": "have fun!"}
]
}
```
## Further Event Methods
Note that the example events covered above cover two different kinds of events:
1. Events that are not specified in the MT Standard (`mt_mint`, `mt_burn`)
2. An event that is covered in the [Multi Token Core Standard](https://nomicon.io/Standards/Tokens/MultiToken/Core#mt-interface). (`mt_transfer`)
This event standard also applies beyond the three events highlighted here, where future events follow the same convention of as the second type. For instance, if an MT contract uses the [approval management standard](https://nomicon.io/Standards/Tokens/MultiToken/ApprovalManagement), it may emit an event for `mt_approve` if that's deemed as important by the developer community.
Please feel free to open pull requests for extending the events standard detailed here as needs arise.
## Reference Implementation
[Minimum Viable Interface](https://github.com/jriemann/near-sdk-rs/blob/multi-token-reference-impl/near-contract-standards/src/multi_token/core/mod.rs)
[MT Implementation](https://github.com/jriemann/near-sdk-rs/blob/multi-token-reference-impl/near-contract-standards/src/multi_token/core/core_impl.rs)
## Copyright
Copyright and related rights waived via [CC0](https://creativecommons.org/publicdomain/zero/1.0/).
[ERC-721]: https://eips.ethereum.org/EIPS/eip-721
[ERC-1155]: https://eips.ethereum.org/EIPS/eip-1155
[storage staking]: https://docs.near.org/concepts/storage/storage-staking
[gas]: https://docs.near.org/concepts/basics/transactions/gas
[MT Metadata]: ../specs/Standards/Tokens/MultiToken/Metadata.md
[NFT Core]: ../specs/Standards/Tokens/NonFungibleToken/Core.md
[Approval Management]: ../specs/Standards/MultiToken/ApprovalManagement.md
[FT Core]: ../specs/Standards/Tokens/FungibleToken/Core.md
[Storage Management]: ../specs/Standards/StorageManagement.md
[MT Enumeration]: ../specs/Standards/Tokens/MultiToken/Enumeration.md
|
Welcome to Day 0 – It’s Just the Beginning
COMMUNITY
October 14, 2020
Welcome to Day 0! It’s been an exciting few days of announcements and even more exciting past 24 hours with Millions of $NEAR being transferred and over 160 Million NEAR Staked.
Echoing our mission to enable community-driven innovation to benefit people around the world, we’re all hands on deck to answer the community’s burning questions about what comes next for NEAR and clarify any questions that have surfaced since transfers were enabled yesterday.
In case you missed it, here’s a recording link of our open community town hall from this morning with members of the NEAR core team live answering community questions.
Here’s a quick roundup of the excitement:
NEAR is Live with Transfers
NEAR is now fully permissionless, decentralized & community-governed. This means it’s now possible for anyone to create accounts, transfer and trade NEAR tokens, and build and launch applications on a performant blockchain that prioritizes ease of use for developers and end users.
Staking & Transfers are Live in the NEAR Wallet!
Earlier this week we released an exciting update to the NEAR Wallet; it now has a staking user interface built directly into the web app. Join the wallet team on Discord in the wallet channel if you have any questions or feedback.
New Foundation Delegation Rewards
With the announcement that Stake Wars was ending, the NEAR Foundation announced three new ways for the community to stay involved with Validators and earn rewards from the NEAR Foundation to promote ecosystem growth.
New Governance Forum
With the official launch of the decentralized community, we created a new open governance forum on our website. Join community conversations on the protocol, the future of decentralization, governance structures on NEAR, and more! Erik kicked us off with a thread about clarifying day 1 supply in circulation and to review Phase II governance in the first governance work group session.
$NEAR is listed on Exchanges
With transfers enabled on chain, open trading and deposits were announced by Binance and Huobi making it possible for new Token holders to join the community.
Applications Live on the NEAR:
We’ve been working closely with the developer community to onboard great applications to NEAR across a variety of use cases like marketplaces, gaming, and NFTs. There are a lot more amazing partners we’ll be announcing in the NEAR future, so stay tuned! For now, here are three exciting applications we’re welcoming to the NEAR Community:
Zed Run – Gaming – a futuristic horse racing universe where users can trade, breed, and race majestic, digital racehorses.
Flux – Open Markets – an open market protocol that enables developers to create markets on anything.
Mintbase – Minting NFTs – Shopify for NFTs, making it easy for anyone to create NFTs.
Updates are coming! Here’s what’s next:
The community came out in full force today to start staking, trading, and using NEAR applications from the moment we went live. This was a huge feat, and something we couldn’t be more excited and thankful for.
As with all product launches, it’s only the beginning. We wanted to take a moment to address some of the common issues we’re hearing from the community and speak to immediate updates planned for the NEAR Wallet on our roadmap that will make using NEAR even easier than before.
Staking in the NEAR Wallet is for Locked Tokens only…for now. Very soon, you’ll be able to stake all tokens, whether locked or unlocked. We built the interface for Locked Tokens first because that represents the vast majority of token holders in the community. This means if your tokens aren’t locked, you can’t use the NEAR Wallet for staking just yet, including viewing any stake positions you have created through Dokia or the command line.
In designing a secure and stable first version of the Wallet, we chose top SMS and Email providers for 2FA. In our coming release, we’ll be optimizing how we deliver through those providers to ensure that any failures to send or lag in delivery won’t affect the end user experience and you’ll receive clear error messages. Currently, there may be a slight delay at times based on your geolocation in receiving an SMS or Email for your 2FA. Tightening partner integrations is a high priority for the team.
We’re working on a bunch of enhancements to how we handle error messages to keep you informed of what’s happening. Keep in mind if you see a scary (or vague) error message, your keys are safe and your Tokens are not lost.
This is especially true for any Recovery issues at the moment. For example, if you see the message “Error: Exceeded maximum attempts for this transaction,” this could be caused by a number of networking issues or simply a slight delay from the RPC nodes. Don’t worry, your keys aren’t lost, try again in a few minutes and it should work.
Staking and Unstaking was the focus of our release Monday and we’re excited to see it being widely used across the community with more than 160 Million NEAR Staked to the network. This participation is what enabled the Validators to pass the vote and move us into Phase 2.
Now that transfers are live and applications are running on NEAR, we are working on improvements to the Unstaking process to allow you to Unstake a partial balance that you may want to trade, transfer, or use in some other way.
Finally, one of the greatest things about NEAR that developers and users will love is the flexible contract-based account model that support many keys per account. This allows you to create very customizable experiences with advanced permissions improving usability for users.
Accounts can be either user-defined (human readable, less than 64 characters) or implicit (derived from a private key, always exactly 64 characters). When creating an account through the NEAR Wallet, your account name will usually be user-defined, and will end in .near. If you use Trust Wallet, or an exchange, they will likely provide you an implicit account to use (Trust Wallet) or deposit to (an exchange). Adding ‘.near’ to the end of an implicit account will result in an error because you will have too many characters in your account name. We’re working on ways to better handle and manage your accounts both ‘.near’ and implicit accounts to make using your accounts on NEAR even easier.
The Wallet team’s roadmap continues to grow and there will be more to come soon. If you have any immediate questions or concerns, join the community discussion on Discord and reach out to the wallet team in their channel.
Join the Community
Once again, thank YOU for being the best part of the NEAR ecosystem and coming with us on this ride. The last few days have been incredibly exciting, but what’s even more exciting is that this is only just the beginning. We’ve got a ton of great partners joining with applications set to launch on NEAR very soon.
If you’re just learning about NEAR for the first time and are seeing this post, we invite you to join us on the journey to make blockchain accessible to everyday people who are excited for the future of the Open Web with NEAR. If you’re a developer, be sure to sign up for our developer program and join the conversation in our Discord chat. You can also keep up to date on future releases, announcements, and event invitations by subscribing to our newsletter for the latest news on NEAR. |
---
id: balance
title: Token Balance
sidebar_label: Token Balance
sidebar_position: 2
---
# Token Balance
Examples from our [RPC](https://docs.near.org/docs/develop/front-end/rpc) are used. If you want to look at information about archival nodes (more than 2 days ago), please use `archival` prefix in your URL (e.g. `https://archival-rpc.mainnet.near.org`) In this article, we always use `POST` methods to the same URL `https://archival-rpc.mainnet.near.org`. Body:
```
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "EXPERIMENTAL_tx_status",
"params": ["8uxq5BAC4zZL4Set7gtraqZSWduYXRUiNggpKhWmBnzK", "nf-finance.near"]
}
```
Every [account](https://docs.near.org/docs/develop/front-end/rpc#view-account) has liquid balance (called `amount`) and stake (called `locked`). All calculation in tables are in yoctonear.
Total supply is the amount of tokens in the whole system. Each example is selected at the moment when no other changes is performed in the blockchain, so the only example could affect total supply.
### Why does my balance change?
There are different scenarios why the amount of tokens could change on the account. Let's look at it on real examples.
Each example (except rewarding) contains fees user pays for the operation. You could read about fees calculation [here](https://nomicon.io/Economics/Economic#transaction-fees).
#### Transfer of tokens
https://explorer.mainnet.near.org/transactions/6gUVYsdAsStzU5H2AyW7bxAdEL4RvJv5PL3b8DRnPVED
`pine.near` sent 1.64234 tokens to `taka.near`
| block height | pine.near amount | taka.near amount | total supply | explanation |
| ------------ | ------------------------- | -------------------------- | ---------------------------------- | -------------------------------------------------------------------------------------------------------- |
| 32786820 | 1997461904089428600000000 | 17799799178272291100000000 | 1020393325841554327182410255742095 | initial state |
| 32786821 | 355076598029241100000000 | 17799799178272291100000000 | 1020393325841554327182410255742095 | pine.near lost some tokens for transferring and fees (with a margin, some tokens will be returned) |
| 32786822 | 355076598029241100000000 | 19442139178272291100000000 | 1020393325841532008926160255742095 | taka.near received exactly 1.64234 tokens. Total supply was deducted for making receipt from transaction |
| 32786823 | 355077267576928600000000 | 19442139178272291100000000 | 1020393325841509690669910255742095 | pine.near received a change. Total supply was deducted for executing receipt |
| 32786824 | 355077267576928600000000 | 19442139178272291100000000 | 1020393325841509690669910255742095 | final state |
For such kind of transactions, sender pays 2 fees: for making receipt from transaction; for executing receipt.
We also have receipt for returning a change: it is made without fee.
For this example, final cost of transferring tokens was `22318256250000000000 * 2 / 10^24 = 0.0000446365125` Near Tokens.
#### Creating the account
[Create Account Receipt](https://explorer.mainnet.near.org/transactions/Hant2anxPJHm3sh8ncc5AWn5VpXTmwq6iyEumVoQcUJP)
`nearcrowd.near` created account `app.nearcrowd.near`, gave the access key, transfer some tokens for that account. These 3 actions are usually go together.
| block height | nearcrowd.near amount | app.nearcrowd.near amount | total supply | explanation |
| ------------ | --------------------------- | --------------------------- | ---------------------------------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------ |
| 32396638 | 117975002547050142700000000 | - | 1019767988635277999737307820909366 | initial state (app.nearcrowd.near does not exist) |
| 32396639 | 17974916362372455200000000 | - | 1019767988635277999737307820909366 | nearcrowd.near spent tokens on transfer and fees (with a margin, some tokens will be returned). Total supply is not changed for now: process is not finished |
| 32396640 | 17974916362372455200000000 | 100000000000000000000000000 | 1019767988635235544231057820909366 | app.nearcrowd.near was created with initial amount of tokens. Total supply was deducted for making receipt from transaction |
| 32396641 | 17974917636037642700000000 | 100000000000000000000000000 | 1019767988635193088724807820909366 | nearcrowd.near received a change. Total supply was deducted for executing receipt |
| 32396642 | 17974917636037642700000000 | 100000000000000000000000000 | 1019767988635193088724807820909366 | final state |
For such kind of transactions, sender pays 2 fees: for making receipt from transaction; for executing receipt.
We also have receipt for returning a change: it is made without fee. For this example, final cost of creating account and transferring tokens were `42455506250000000000 * 2 / 10^24 = 0.0000849110125` Near Tokens.
#### Deleting the account
[Delete Account Receipt](https://explorer.mainnet.near.org/transactions/8nZeedU6RUnj65zxQYHrGx2Urx5sLe6J2T123xSNL2RK)
It's not possible to delete not your account: user is always deleting their own account. User should provide beneficiary account that receive all tokens. User could provide the account that does not exist, in such case the tokens will burn.
`wxx.near` deleted their account with beneficiary `vng.near`
| block height | wxx.near amount | vng.near amount | total supply | explanation |
| ------------ | ------------------------ | ------------------------- | ---------------------------------- | -------------------------------------------------------------------------------------- |
| 31961454 | 456172376354355113240646 | 719560525627200400000000 | 1019079411898934110106918776083373 | initial state |
| 31961455 | - | 719560525627200400000000 | 1019079411898934110106918776083373 | wxx.near was deleted |
| 31961456 | - | 1175681792281555513240646 | 1019079411898883000406918776083373 | vng.near received tokens (minus fee), total supply was deducted by fees getting burned |
| 31961457 | - | 1175681792281555513240646 | 1019079411898883000406918776083373 | final state |
For such kind of transactions, sender pays 2 fees: for making receipt from transaction; for executing receipt.
Note: we do not have the change. For this example, final cost of deleting account was `25554850000000000000 * 2 / 10^24 = 0.0000511097` Near Tokens.
#### Calling a function
[Function Call Receipt](https://explorer.mainnet.near.org/transactions/8oBZrKk8jkAzrsYasoL2DW9Yg6K2GznLhvoiXmSm7kHe)
`relayer.bridge.near` invoked a function in the contract of `client.bridge.near`
| block height | relayer.bridge.near amount | client.bridge.near amount | total supply | explanation |
| ------------ | --------------------------- | ---------------------------- | ---------------------------------- | ----------------------------------------------------------------------------------------------------- |
| 32451005 | 927695372457390994900000000 | 1018709186646522709500000000 | 1019836940956022237114094657887001 | initial state |
| 32451006 | 927676238135400182753029324 | 1018709186646522709500000000 | 1019836940956022237114094657887001 | the function is invoked, relayer.bridge.near spent the tokens on the fees (with a margin) |
| 32451007 | 927676238135400182753029324 | 1018710176966066945800000000 | 1019836940955771421812122457887001 | author of the function gets the reward. Total supply was deducted for making receipt from transaction |
| 32451008 | 927691569761639596000000000 | 1018710176966066945800000000 | 1019836940953209860906932057887001 | relayer.bridge.near received a change for fees. Total supply was deducted for executing receipt |
| 32451009 | 927691569761639596000000000 | 1018710176966066945800000000 | 1019836940953209860906932057887001 | final state |
For such kind of transactions, sender pays 2 fees: for making receipt from transaction; for executing receipt. Receiver gets part of the fee as a reward for a possibility to invoke their function.
For this example, final cost of invoking the function was `(250815301972200000000 + 3551880449426700000000) / 10^24 = 0.0038026957513989` Near Tokens. Reward was `0.0009903195442363` Near Tokens.
<!-- TODO: add Nomicon link about royalties when [this issue](https://github.com/near/NEPs/issues/198) will be resolved. -->
<!-- **The example of calling a function could be more complex.** -->
[Transaction Receipt](https://explorer.mainnet.near.org/transactions/DuGWWTK2sAxjffifJiJBv6hs3Hc8MdhpHnzgRJryV4Be)
`lulukuang.near` invokes a function in a protocol of `berryclub.ek.near`. It triggers `berryclub.ek.near` to send the money to third account, `farm.berryclub.ek.near`. So we need to analyze 3 accounts in this scenario.
| block height | lulukuang.near amount | berryclub.ek.near amount | farm.berryclub.ek.near amount | total supply |
| ------------ | -------------------------- | --------------------------- | ----------------------------- | ---------------------------------- |
| 32448898 | 13565822561282329011544599 | 468266310630308773724199697 | 1795104370794561556009708395 | 1019836941925593000345074757887001 |
| 32448899 | 13553154814244264373824919 | 468266310630308773724199697 | 1795104370794561556009708395 | 1019836941925593000345074757887001 |
| 32448900 | 13553154814244264373824919 | 468011594500695874498002337 | 1795104370794561556009708395 | 1019836941925350190357602757887001 |
| 32448901 | 13563348945268324922643655 | 468011594500695874498002337 | 1795359347718068910135905755 | 1019836941924550160945561757887001 |
#### Adding the key
[Add Key Receipt](https://explorer.mainnet.near.org/transactions/A9767GbmRCLdeCpZYfKTQUqe17KyAu5JrZHanoRChMSV)
`slavon.near` added the key to their own account.
| block height | slavon.near amount | total supply | explanation |
| ------------ | ------------------------- | ---------------------------------- | ------------------------- |
| 32452281 | 4110095852838015413724607 | 1019836940258537920949718457887001 | initial state |
| 32452282 | 4110053774694109613724607 | 1019836940258537920949718457887001 | fees are gone |
| 32452283 | 4110053774694109613724607 | 1019836940258495842805812657887001 | total supply was deducted |
| 32452284 | 4110053774694109613724607 | 1019836940258495842805812657887001 | final state |
For such kind of transactions, sender pays 2 fees: for making receipt from transaction; for executing receipt.
Note: we do not have the change. For this example, final cost of adding the key was `21039071952900000000 * 2 / 10^24 = 0.0000420781439058` Near Tokens.
#### Deleting the key
[Delete Key Receipt](https://explorer.mainnet.near.org/transactions/CfuXcVPy7vZNVabYKzemSwDdNF9fnhbMLaaDKjAMyw8j)
`77yen.near` deleted the key from their own account.
| block height | 77yen.near amount | total supply | explanation |
| ------------ | ------------------------ | ---------------------------------- | ------------------------- |
| 32429428 | 350892032360972200000000 | 1019836950055687104230777157887001 | initial state |
| 32429429 | 350851431135972200000000 | 1019836950055687104230777157887001 | fees are gone |
| 32429430 | 350851431135972200000000 | 1019836950055646503005777157887001 | total supply was deducted |
| 32429431 | 350851431135972200000000 | 1019836950055646503005777157887001 | final state |
For such kind of transactions, sender pays 2 fees: for making receipt from transaction; for executing receipt.
Note: we do not have the change. For this example, final cost of adding the key was `20300612500000000000 * 2 / 10^24 = 0.000040601225` Near Tokens.
#### Deploying the contract
[Deployment Receipt](//explorer.mainnet.near.org/transactions/3DN4XiQCX2EeSN5sjiaB4WBjJizthbhUfqQhCBcZK57A)
`ref-finance.near` deployed the contract.
| block height | ref-finance.near amount | total supply | explanation |
| ------------ | -------------------------- | ---------------------------------- | ------------------------- |
| 32378845 | 42166443062029468200000000 | 1019767995481527182962669020909366 | initial state |
| 32378846 | 42165929455413658400000000 | 1019767995481527182962669020909366 | fees are gone |
| 32378847 | 42165929455413658400000000 | 1019767995481013576346859220909366 | total supply was deducted |
| 32378848 | 42165929455413658400000000 | 1019767995481013576346859220909366 | final state |
For such kind of transactions, sender pays 2 fees: for making receipt from transaction; for executing receipt.
Note: we do not have the change. For this example, final cost of deploying the contract was `256803307904900000000 * 2 / 10^24 = 0.0005136066158098` Near Tokens.
#### Receiving the reward for being validator
NB: No transaction/receipt is associated with such type of balance changing.
On the boundary of the epochs, validators receive reward. The platform gives 10% of minted tokens to special account `treasury.near`. You could think about `treasury.near` as about reward to the whole system to keep it in working order. Total sum of the rewards (including `treasury.near`) is the increase of total supply in the system.
| | total supply | treasury.near | comment |
| -------------- | ---------------------------------- | ------------------------------- | ------------------------------- |
| block 32716252 | 1020253586314335829144818854680815 | 2047777174062240806436499682917 | |
| block 32716253 | 1020323466696960098722157540903707 | 2054765212324667764170368305209 | |
| difference | 69880382624269577338686222892 | 6988038262426957733868622292 | treasury received 10% of reward |
If the validator does not change the stake during the epoch, all the reward will go to their stake. Liquid balance will remain the same.
| block height | baziliknear.poolv1.near amount | baziliknear.poolv1.near locked (stake) |
| ------------ | ------------------------------ | -------------------------------------- |
| 32716252 | 87645929417382101548688640817 | 4051739369484142981303684413288 |
| 32716253 | 87645929417382101548688640817 | 4052372556336355400023161624996 |
`baziliknear.poolv1.near` had 4M tokens on their locked balance. Their reward for the epoch was `633186852212418719477211708 / 10^24 = 633.1868522124187` tokens.
If the validator decrease the stake during the epoch, it will be actually decreased at the boundary between the epochs. The reward will also go to the liquid balance in such case.
| block height | astro-stakers.poolv1.near amount | astro-stakers.poolv1.near locked (stake) | d1.poolv1.near amount | d1.poolv1.near locked (stake) | artemis.poolv1.near amount | artemis.poolv1.near locked (stake) |
| -------------- | -------------------------------- | ---------------------------------------- | ----------------------------- | ------------------------------- | ------------------------------- | ---------------------------------- |
| block 32716252 | 75643656264518222662518079298 | 14871875685703104705137979278533 | 39579636061549418675704317463 | 9002215926886116642974675441492 | 195455191635613196116221250 | 3155647821138218410536743286795 |
| block 32716253 | 77968865959341187332341390150 | 14871875685703104705137979278533 | 40986873900034981089977167653 | 9002215926314400176270075441492 | 3155843276329854023732859508045 | 0 |
| diff | 2325209694822964669823310852 | 0 | 1407237838485562414272850190 | -571716466704600000000 | 3155647821138218410536743286795 | -3155647821138218410536743286795 |
`astro-stakers.poolv1.near` decided to put the reward to liquid balance, so they invoked a command (e.g. `ping`). We can see that the stake does not change between the epochs.
`d1.poolv1.near` decided to slightly decrease the stake size, so the reward and the part of the stake are on the liquid balance.
`artemis.poolv1.near` received the reward and stopped being validator at that epoch, so all the stake are on the liquid balance, stake becomes zero.
The reword value is calculated based on stake size and number of calculated blocks per epoch. Read more about it on the [Economics](https://nomicon.io/Economics/Economic#validator-rewards-calculation) page of the Nomicon.
If the validator increase the stake during the epoch, stake will be increased in next few blocks, we do not need to wait for the moment of the epoch boundary. For current epoch, blockchain will take into account stake value fixed at the start of the epoch.
[Transaction Receipt](https://explorer.mainnet.near.org/transactions/HajXjY1gSf47jakXP44ERecjFE2hFvF4FgRyQzNdZzDZ)
`elecnodo.near` put 245.7788678019393 tokens from its locked account `57bea511f28a9c0d44148c332826a9b9fb14f13c.lockup.near` to a staking pool `staked.poolv1.near`
Read more about epochs changing in the [NEP](https://github.com/near/NEPs/tree/master/specs/BlockchainLayer/EpochManager) guide.
|
NEARCON ‘23 Day Two Begins: Expand Your Open Web Horizons
NEAR FOUNDATION
November 9, 2023
The second day of NEARCON 23 is kicking off with an awesome agenda, carrying over the massive momentum from Day One. Doors fling wide open at 9:00 AM for all attendees, so make sure to grab a coffee and a quick breakfast bite before the sessions really get ramped up at 10:45 AM all the way through 5:30 PM.
You won’t want to miss a moment of Day Two, so read on for a complete rundown.
Join the morning brunch at Community HQ
This year, we’re paying tribute to the dynamic women shaping the Web3 ecosystem, and leading a discussion on how to nurture the next generation of top talent. Join us for the Women in Web3 Changemakers breakfast at Community HQ at 8:30 AM. It’s a must attend event for anyone wishing to learn about the challenges and career opportunities available to women — and how to make the World of Web3 brighter for all.
Can’t miss sessions on Day Two
Day Two at NEARCON ‘23 will dive into the intersection of the open web and traditional industries and explore new developments in digital identity, including a must see fireside chat with NEAR CEO Illia Polosukhin and TechCrunch.
SK Telecom’s Leap Into Web3 (11:25 AM – 11:40 AM, Layer 1 Stage): Sehyeon Oh, EVP at SK Telecom, unveils the telecom giant’s Web3 strategy, including an open web wallet and NFT marketplace initiative. Learn about how a traditional industry is collaborating with NEAR to expand the reach of the open web..
AI x Blockchain: Illia Polosukhin and Mike Butcher in Conversation (3:50 PM – 4:15 PM, Layer 1 Stage): Join Illia Polosukhin, NEAR co-founder, and Mike Butcher of TechCrunch as they dissect the interplay between AI and Web3, and what the future holds for their convergence.
From Nightshade to Starsight: The Evolution of NEAR Protocol Design (2:40 PM – 3:00 PM, Layer 2 Stage): Pagoda’s Bowen Wang provides insights into NEAR Protocol’s recent advancements and teases upcoming developments regarding protocol design and the next evolution of sharding.
Introducing Decentralized Identity Solutions With the idOS (1:45 PM – 2:15 PM, Block Zero Stage): Julian Leitloff explains how the Identity Operating System (idOS) is transforming digital identity, offering users sovereign control over their personal data with their pioneering open-source DID solution.
More Speaker Highlights
Hiring Wisdom for Web3 (11:00 AM – 11:15 AM, Layer 2 Stage): Dan Eskow, open web recruiter at Up Top, shares invaluable hiring tactics tailored for the dynamic Web3 sector, where finding the right fit is as challenging as it is critical.
Economic Design in Protocols (11:45 AM – 12:00 PM, Layer 2 Stage): Lisa JY Tan from Economics Design guides us through the intricacies of creating economically sound and sustainable protocols, offering vital insights for VCs and entrepreneurs.
Bug Bounties and Web3 Security (3:30 PM – 3:45 PM, Layer 2 Stage): Mitchell Amador, founder and CEO of Immunefi, narrates the journey to establishing the most substantial bug bounties in the open web.
Strategizing DAO Resource Allocation (4:25 PM – 4:40 PM, Layer 2 Stage): Andre Geest of Safe discusses optimizing resource distribution within DAOs, presenting a balanced approach to boost both governance and community engagement.
Founders Forum and IRL Hackathon
For entrepreneurs forging the next wave of open innovation, the Founders Forum at Community HQ is the place to be. Dive into sessions from 11:30 AM to 5:00 PM that dissect critical legal and regulatory aspects for startups, explore the equilibrium between decentralization and compliance, and uncover strategies for assembling a stellar team in the Web3 domain.
Also, a reminder for all Hackathon participants. Finalize your projects by the 5:00 PM deadline and prepare to showcase your open web ingenuity.
Sunset socializing and networking
After the jam-packed sessions all morning and afternoon, get ready to wind down and relax before calling it a day — or getting revved up for a late Lisbon night.
Movers and Shakers Happy Hour (5pm – 7pm, Community HQ): Mingle with your newest NEARCON friends and connections at an epic happy hour sponsored by Glass and Moonbounce.
SailGP Happy Hour (5:45 pm – 7pm, NEARCON HQ): Swap stories and fresh insights right outside the Layer 2 Stage as the final session ends. Who knows, you might even score a collab or two while you’re at it.
Shout out to the Day Two sponsors
A heartfelt thank you to today’s sponsors, without whose all-out support such an event would not have been possible.
Glass
Moonbounce
SailGP
Get ready for a day brimming with Web3 wisdom, open web masterminds, and the chance to engage with the brightest and bravest of the NEAR ecosystem. It’s a unique concoction of insight, education, and fun that only NEARCON ‘23 can cook up. So seize the day, connect at the happy hours, and let’s make Day Two a milestone for the entire open web.
|
NEAR and Alibaba Cloud Team Up to Grow the Open Web
NEAR FOUNDATION
June 26, 2023
NEAR Foundation is excited to announce a major new partnership with Alibaba Cloud, the digital technology and intelligence backbone of Alibaba Group.
In this new partnership, NEAR Foundation will collaborate with Alibaba Cloud to offer Remote Procedure Calls (RPC) as a service to developers and users in the NEAR ecosystem, as well as multi-chain data indexing to provide data query API to developers. The partnership will also find the companies collaborating on tools to help developers build decentralized applications more easily.
“Our partnership with Alibaba Cloud is a major milestone for the NEAR Foundation as it proves that NEAR protocol’s technology has the capabilities to truly lift the restrictions surrounding Web3 development to allow more creators to build exciting applications while also helping to onboard billions of Web2 users to the possibility of Web3.,” said Marieke Flament, CEO of the NEAR Foundation. “We look forward to supporting Alibaba Cloud as it leverages the scalability of NEAR Blockchain and the BOS to expand its Web3 offerings to a global market.”
Alibaba Cloud infrastructure to help power the BOS
App developers building on the NEAR Blockchain Operating System (BOS) — a common layer for browsing and discovering open web experiences, compatible with any blockchain — will now also enjoy Alibaba Cloud’s infrastructure.
With BOS, now accessible directly on Near.org through a browser, developers can easily fork components and create new experiences, while end users can explore open web experiences, social, and news all in one place. FastAuth, a new standout BOS feature, allows app developers to onboard new users with only an email — no crypto required.
Alibaba Cloud’s plug-and-play infrastructure will empower NEAR developers
Commenting on the partnership, Raymond Xiao, Head of International Web3 Solutions, Alibaba Cloud Intelligence, said: “The NEAR Foundation and Alibaba Cloud partnership is an important one as we continue to support Web3 developers to explore opportunities.”
“It is also significant for developers and validators in the Asian markets, as they can leverage Alibaba Cloud’s comprehensive infrastructure in Asia,” Xioa added. “Together with NEAR’s Node-as-a-Service initiative and BOS, we supports the Web3 community create exciting applications that will benefit a wide consumer audience. We are looking forward to what we can achieve with this partnership.”
NEAR Foundation and Alibaba Cloud will continue to expand its partnership together through collaborations at a variety of global Web3 conferences and events. |
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
<Tabs groupId="nft-contract-tabs" className="file-tabs">
<TabItem value="NFT Primitive" label="Reference" default>
```bash
near view nft.primitives.near nft_token '{"token_id": "1"}'
```
<details>
<summary> Example response </summary>
```json
{
"token_id": "1",
"owner_id": "bob.near",
"metadata": {
"title": "string", // ex. "Arch Nemesis: Mail Carrier" or "Parcel #5055"
"description": "string", // free-form description
"media": "string", // URL to associated media, preferably to decentralized, content-addressed storage
"media_hash": "string", // Base64-encoded sha256 hash of content referenced by the `media` field. Required if `media` is included.
"copies": 1, // number of copies of this set of metadata in existence when token was minted.
"issued_at": 1642053411068358156, // When token was issued or minted, Unix epoch in milliseconds
"expires_at": 1642053411168358156, // When token expires, Unix epoch in milliseconds
"starts_at": 1642053411068358156, // When token starts being valid, Unix epoch in milliseconds
"updated_at": 1642053411068358156, // When token was last updated, Unix epoch in milliseconds
"extra": "string", // anything extra the NFT wants to store on-chain. Can be stringified JSON.
"reference": "string", // URL to an off-chain JSON file with more info.
"reference_hash": "string" // Base64-encoded sha256 hash of JSON from reference field. Required if `reference` is included.
}
}
```
</details>
</TabItem>
<TabItem value="Paras" label="Paras">
```bash
near view x.paras.near nft_token '{"token_id": "84686:1154"}'
```
<details>
<summary> Example response </summary>
```json
{
"token_id": "84686:1154",
"owner_id": "bob.near",
"metadata": {
"title": "Tokenfox Silver Coin #1154",
"description": null,
"media": "bafkreihpapfu7rzsmejjgl2twllge6pbrfmqaahj2wkz6nq55c6trhhtrq",
"media_hash": null,
"copies": 4063,
"issued_at": "1642053411068358156",
"expires_at": null,
"starts_at": null,
"updated_at": null,
"extra": null,
"reference": "bafkreib6uj5kxbadfvf6qes5flema7jx6u5dj5zyqcneaoyqqzlm6kpu5a",
"reference_hash": null
},
"approved_account_ids": {}
}
```
</details>
</TabItem>
<TabItem value="Mintbase" label="Mintbase">
```bash
near view anthropocene.mintbase1.near nft_token '{"token_id": "17960"}'
```
<details>
<summary> Example response </summary>
```json
{
"token_id": "17960",
"owner_id": "876f40299dd919f39252863e2136c4e1922cd5f78759215474cbc8f1fc361e14",
"approved_account_ids": {},
"metadata": {
"title": null,
"description": null,
"media": null,
"media_hash": null,
"copies": 1,
"issued_at": null,
"expires_at": null,
"starts_at": null,
"updated_at": null,
"extra": null,
"reference": "F-30s_uQ3ZdAHZClY4DYatDPapaIRNLju41RxfMXC24",
"reference_hash": null
},
"royalty": {
"split_between": {
"seventhage.near": {
"numerator": 10000
}
},
"percentage": {
"numerator": 100
}
},
"split_owners": null,
"minter": "anthropocene.seventhage.near",
"loan": null,
"composeable_stats": { "local_depth": 0, "cross_contract_children": 0 },
"origin_key": null
}
```
</details>
:::note
When someone creates a NFT on Mintbase they need to deploy their own NFT contract using Mintbase factory. Those smart contract are subaccounts of mintbase1.near, e.g. `anthropocene.mintbase1.near`.
:::
</TabItem>
</Tabs>
|
# Enumeration
## [NEP-181](https://github.com/near/NEPs/blob/master/neps/nep-0181.md)
Version `1.0.0`
## Summary
Standard interfaces for counting & fetching tokens, for an entire NFT contract or for a given owner.
## Motivation
Apps such as marketplaces and wallets need a way to show all tokens owned by a given account and to show statistics about all tokens for a given contract. This extension provides a standard way to do so.
While some NFT contracts may forego this extension to save [storage] costs, this requires apps to have custom off-chain indexing layers. This makes it harder for apps to integrate with such NFTs. Apps which integrate only with NFTs that use the Enumeration extension do not even need a server-side component at all, since they can retrieve all information they need directly from the blockchain.
Prior art:
- [ERC-721]'s enumeration extension
## Interface
The contract must implement the following view methods:
```ts
// Returns the total supply of non-fungible tokens as a string representing an
// unsigned 128-bit integer to avoid JSON number limit of 2^53; and "0" if there are no tokens.
function nft_total_supply(): string {}
// Get a list of all tokens
//
// Arguments:
// * `from_index`: a string representing an unsigned 128-bit integer,
// representing the starting index of tokens to return
// * `limit`: the maximum number of tokens to return
//
// Returns an array of Token objects, as described in Core standard, and an empty array if there are no tokens
function nft_tokens(
from_index: string|null, // default: "0"
limit: number|null, // default: unlimited (could fail due to gas limit)
): Token[] {}
// Get number of tokens owned by a given account
//
// Arguments:
// * `account_id`: a valid NEAR account
//
// Returns the number of non-fungible tokens owned by given `account_id` as
// a string representing the value as an unsigned 128-bit integer to avoid JSON
// number limit of 2^53; and "0" if there are no tokens.
function nft_supply_for_owner(
account_id: string,
): string {}
// Get list of all tokens owned by a given account
//
// Arguments:
// * `account_id`: a valid NEAR account
// * `from_index`: a string representing an unsigned 128-bit integer,
// representing the starting index of tokens to return
// * `limit`: the maximum number of tokens to return
//
// Returns a paginated list of all tokens owned by this account, and an empty array if there are no tokens
function nft_tokens_for_owner(
account_id: string,
from_index: string|null, // default: 0
limit: number|null, // default: unlimited (could fail due to gas limit)
): Token[] {}
```
## Notes
At the time of this writing, the specialized collections in the `near-sdk` Rust crate are iterable, but not all of them have implemented an `iter_from` solution. There may be efficiency gains for large collections and contract developers are encouraged to test their data structures with a large amount of entries.
[ERC-721]: https://eips.ethereum.org/EIPS/eip-721
[storage]: https://docs.near.org/concepts/storage/storage-staking
|
Check Out the Pagoda Product Roadmap
DEVELOPERS
October 19, 2023
Over the past year, Pagoda has been working hard to build out the B.O.S, evolve near.org, and continue developing the NEAR protocol. While Pagoda has built and launched proprietary services in the past, almost all of our work so far in 2023 and planned for the future is free open-source software, which we intend to become vital parts of the NEAR ecosystem. We want the community to be aware of, excited by, and engaged in this work.
So I’m excited to announce that Pagoda is making its product roadmap public this week via GitHub. It’s honestly a bit overdue. Given our values of transparency and collaboration, we hope to involve more members of the community in the development process. We also hope this lets teams across the NEAR ecosystem plan ahead a bit better, build in parallel to Pagoda, and even get ideas for what to build based on what you feel is missing or should be prioritized.
Right now this roadmap covers the rest of 2023 and we are committing to update the roadmap at least quarterly. The roadmap on GitHub is arranged into several views covering both the company objectives (OKRs) as well as larger workstreams (initiatives). We’ve further organized our roadmap views by product vertical and priority level, along with status and expected delivery date (which the product leads will update in real time). You may see some roadmap items displayed as “private”; this is because certain partnerships and enterprise workstreams must remain under NDA or embargo until launch. The Pagoda team will continue to iterate on this roadmap tool to make it an even richer resource for ecosystem partners and the NEAR community.
Delivering on the Open Web vision will take contributions far beyond Pagoda and we look forward to working alongside those who are as passionate about NEAR as we are. Please explore and leave comments or questions on the NEAR Social post – or on the roadmap itself – if you have feedback. |
---
id: contract
title: Contract
sidebar_label: Contract
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
When you instantiate an instance of `Contract` you need to specify the names of the functions you have on your smart contract.
Then the new instance of `Contract` will have methods with the same names as your smart contract functions.
For example if you deployed a contract with `my_smart_contract_function` function on it, then this will work:
```js
const contract = new Contract(account, "example-contract.testnet", {
changeMethods: ["my_smart_contract_function"], // your smart-contract has a function `my_smart_contract_function`
});
// `contract` object has `my_smart_contract_function` function on it:
contract.my_smart_contract_function();
```
### Load Contract {#load-contract}
<Tabs>
<TabItem value="Standard" label="Standard" default>
```js
const { Contract } = nearAPI;
const contract = new Contract(
account, // the account object that is connecting
"example-contract.testnet",
{
// name of contract you're connecting to
viewMethods: ["getMessages"], // view methods do not change state but usually return a value
changeMethods: ["addMessage"], // change methods modify state
}
);
```
[<span className="typedoc-icon typedoc-icon-class"></span> Class `Contract`](https://near.github.io/near-api-js/classes/_near_js_accounts.contract.Contract.html)
</TabItem>
<TabItem value="wallet" label="Using Wallet">
```js
const { Contract } = nearAPI;
const contract = new Contract(
wallet.account(), // the account object that is connecting
"example-contract.testnet",
{
// name of contract you're connecting to
viewMethods: ["getMessages"], // view methods do not change state but usually return a value
changeMethods: ["addMessage"], // change methods modify state
}
);
```
[<span className="typedoc-icon typedoc-icon-class"></span> Class `Contract`](https://near.github.io/near-api-js/classes/_near_js_accounts.contract.Contract.html)
</TabItem>
</Tabs>
### Call Contract {#call-contract}
<Tabs>
<TabItem value="method" label="Change Method" default>
```js
const contract = new Contract(account, "example-contract.testnet", {
changeMethods: ["method_name"],
});
await contract.method_name(
{
arg_name: "value", // argument name and value - pass empty object if no args required
},
"300000000000000", // attached GAS (optional)
"1000000000000000000000000" // attached deposit in yoctoNEAR (optional)
);
```
</TabItem>
<TabItem value="callback" label="Change Method w/ callbackUrl and meta">
```js
const contract = new Contract(account, "example-contract.testnet", {
changeMethods: ["method_name"],
});
await contract.method_name({
callbackUrl: "https://example.com/callback", // callbackUrl after the transaction approved (optional)
meta: "some info", // meta information NEAR Wallet will send back to the application. `meta` will be attached to the `callbackUrl` as a url param
args: {
arg_name: "value", // argument name and value - pass empty object if no args required
},
gas: 300000000000000, // attached GAS (optional)
amount: 1000000000000000000000000, // attached deposit in yoctoNEAR (optional)
});
```
</TabItem>
<TabItem value="view" label="View Method">
```js
const contract = new Contract(account, "example-contract.testnet", {
viewMethods: ["view_method_name"],
});
const response = await contract.view_method_name();
```
</TabItem>
<TabItem value="args" label="View Method w/ args">
```js
const contract = new Contract(account, "example-contract.testnet", {
viewMethods: ["view_method_name"],
});
const response = await contract.view_method_name({ arg_name: "arg_value" });
```
</TabItem>
</Tabs>
[<span className="typedoc-icon typedoc-icon-class"></span> Class `Contract`](https://near.github.io/near-api-js/classes/_near_js_accounts.contract.Contract.html)
[//]: # "## Transactions {#transactions}"
[//]: # "A [Transaction](/concepts/protocol/transactions) is a collection of Actions, and there are few types of Actions."
[//]: # "For every type of Action there is a function on Account that you can use to invoke the Action, but Account also exposes `signAndSendTransaction` function which you can use to build and invoke a batch transaction."
|
NEAR Q4: Hackathons, Upgrades and Integrations
COMMUNITY
December 15, 2021
It’s been quite a year here at NEAR, and it’s been an even better Q4. There’s been so much activity, we thought we’d provide a quick snapshot of what’s been happening in and around the ecosystem. We’ve got upgrades, new projects, partnerships, meetups, the works.
Metabuild, take-two
NEAR is thriving! Here is a snapshot of all the things that have happened in Q4 of 2021.
Metabuild is back! The first NEAR Metabuild took place earlier this year, and was all about the metaverse! We gave away $1 million in prize funds to projects, but this time we’re going even bigger. We’re looking for people at the very beginning of their crypto journey to bring their ideas for storytelling and education, as well developers looking to build their first app on Web3. On top of that, we have our crypto natives track, where we’re on the hunt for NEAR, Aurora, and Octopus developers building incredible ideas around DeFi, Web3, Gaming, Digital Art/Collectibles, Migration, Ecosystem, and tools. Oh and there’s $1 million in prizes to be awarded.
deadmau5 x Portugal. The Man x Mintbase Launches and NFT
Mintbase, the next generation NFT utility engine announced a partnership with DJ and producer deadmau5 and rock band Portugal. The Man to release their new single as an NFT. The song, titled “this is fine” is being sold as a collection of 1 million NFTs. By releasing the song as an NFT, deadmau5, and Portugal. The Man could be the first artists to reach platinum status – selling one million copies in the US – with an NFT single release. Tooling UpdatesProtocol Upgrades
Protocol Upgrades
The NEAR protocol went through 20 successful upgrades in 2021. This included bug fixes, improvements to performance, and changes to help improve usability and efficiency.
Among the highlights were protocol update 35 – implicit accounts. This allows NEAR to have Ethereum style accounts that are public keys. They are created on-chain when someone transfers a nonzero amount of tokens to this account.
Another stand-out upgrade was protocol update 42. Here engineers lowered storage staking cost from 1N per 10kb to 1N per 100kb. This dramatically reduced the barrier of entry for contract developers. Before this change, when a developer deployed a 300kb contract, they needed to have at least 30 $NEAR on the account. After the change, they only need 3 $NEAR.
Lastly, protocol upgrade 48 brought our WebAssembly runtime from wasmer0 to wasmer2. This shift helped half the cost of wasm operations, helping unlock many applications building on Aurora that were previously blocked because their transactions could not fit in NEAR’s gas limit.
This trend continued throughout the year, culminating in our biggest protocol announcement this year, Sharding.
Sharding is Here
In November, the NEAR network was successfully upgraded to become a sharded blockchain! This phase of the product roadmap, nicknamed Simple Nightshade, will kickstart a year of changes and additions to the NEAR blockchain that when complete, will make it super-fast, incredibly secure, and capable of onboarding millions of users into the world of Web3 without skipping a beat.
Aurora Integrations
Aurora, the scaling solution that allows projects on Ethereum to utilize NEAR’s cutting-edge technology has had a busy quarter! Not only did it successfully launch its token on mainnet, but it has also announced a number of strategic partnerships.
The first, brought Chainlink’s price feeds to enable rapid, EVM-compatible DeFi development.
Next up was a partnership with ConsenSys. This partnership brings products like MetaMask, Infura, and Codefi to the NEAR ecosystem. Shortly after that, Aurora teamed up The Graph to help bring superior data indexing.
Lastly, but not leastly, Aurora has partnered with another ecosystem project, Flux Protocol, to bring decentralized oracles to the NEAR ecosystem.
MoonPay Takes NEAR worldwide
NEAR took a giant leap forward in its goal to be the on-ramp for the world into web3 with a new partnership with MoonPay. From December, people in 150 countries worldwide, including the United States, will be able to buy NEAR using their credit or debit cards in just a few clicks.
Flux Goes Live
In Q4 this year, another major new project launched on NEAR Mainnet. Flux is an oracle from the future. Completely decentralized, Flux is bringing secured data feeds to any and all high-throughput data apps — like trading platforms that need access to lots of reliable data — on the NEAR blockchain. Flux’s unique feature set will bring a next-generation oracle capable of powering mainstream use cases like derivatives, lending, stablecoins, dynamic NFTs, yield farming, reserve currencies, insurance, asset bridging, off-chain computation, and more to NEAR.
|
- Proposal Name: Improve view/change methods in contracts
- Start Date: 2019-09-26
- NEP PR: [nearprotocol/neps#0000](https://github.com/nearprotocol/neps/pull/18)
# Summary
[summary]: #summary
Currently the separation between view methods and change methods on the contract level is not very well defined and causes
quite a bit of confusion among developers. We propose in the NEP to elucidate the difference between view methods
and change methods and how they should be used. In short, we would like to restrict view methods from accessing certain
context variables and do not distinguish between view and change methods on the contract level. Developers have the option
to differentiate between the two in frontend or through near-shell.
# Motivation
[motivation]: #motivation
From the feedback we received it seems that developers are confused by the results they get from view calls, which are
mainly caused by the fact that some binding methods such as `signer_account_id`, `current_account_id`, `attached_deposit`
do not make sense in a view call.
To avoid such confusion and create better developer experience, it is better if those context variables
are prohibited in view calls.
# Guide-level explanation
[guide-level-explanation]: #guide-level-explanation
Among binding methods that we expose from nearcore, some do make sense in a view call, such as `block_index`,
while the majority does not.
Here we explicitly list the methods are not allowed in a view call and, in case they are invoked, the contract will panic with
`<method_name> is not allowed in view calls`.
The following methods are prohibited:
* `signer_account_id`
* `signer_account_pk`
* `predecessor_account_id`
* `attached_deposit`
* `prepaid_gas`
* `used_gas`
* `promise_create`
* `promise_then`
* `promise_and`
* `promise_batch_create`
* `promise_batch_then`
* `promise_batch_action_create_account`
* `promise_batch_action_deploy_account`
* `promise_batch_action_function_call`
* `promise_batch_action_transfer`
* `promise_batch_action_stake`
* `promise_batch_action_add_key_with_full_access`
* `promise_batch_action_add_key_with_function_call`
* `promise_batch_action_delete_key`
* `promise_batch_action_delete_account`
* `promise_results_count`
* `promise_result`
* `promise_return`
From the developer perspective, if they want to call view functions from command line on some contract, they would just
call `near view <contractName> <methodName> [args]`. If they are building an app and want to call a view function from the
frontend, they should follow the same pattern as we have right now, specifying `viewMethods` and `changeMethods` in
`loadContract`.
# Reference-level explanation
[reference-level-explanation]: #reference-level-explanation
To implement this NEP, we need to change how binding methods are handled in runtime. More specifically, we can rename
`free_of_charge` to `is_view` and use that to indicate whether we are processing a view call. In addition we can add
a variant `ProhibitedInView(String)` to `HostError` so that if `is_view` is true,
then all the access to the prohibited
methods will error with `HostError::ProhibitedInView(<method_name>)`.
# Drawbacks
[drawbacks]: #drawbacks
In terms of not allowing context variables, I don't see any drawback as those variables do not have a proper meaning
in view functions. For alternatives, see the section below.
# Rationale and alternatives
[rationale-and-alternatives]: #rationale-and-alternatives
This design is very simple and requires very little change to the existing infrastructure. An alternative solution is
to distinguish between view methods and change methods on the contract level. One way to do it is through decorators, as
described [here](https://github.com/nearprotocol/NEPs/pull/3). However, enforcing such distinction on the contract level
requires much more work and is not currently feasible for Rust contracts.
# Unresolved questions
[unresolved-questions]: #unresolved-questions
# Future possibilities
[future-possibilities]: #future-possibilities
|
NEAR x CoinGecko Raffle Winner: Terms and Conditions
NEAR FOUNDATION
May 17, 2023
Introduction:
The following terms and conditions (the “Terms”) govern the use of the NEAR Foundation Raffle (the “Raffle”) provided by NEAR Foundation (“we,” “us,” “our” or the “Company”). By participating in the Raffle, you (“you” or “Participant”) agree to be bound by these Terms. If you do not agree to these Terms, you may not be eligible to participate in the Raffle or receive any rewards. Note: the Raffle is in conjunction with CoinGecko’s NFT giveaway. Terms of the NFT giveaway can be found here.
Eligibility:
The Raffle is available only to individuals who are at least 18 years old. By participating in the Raffle, you represent and warrant that you are at least 18 years old.
Participation in the Raffle:
We are excited to collaborate with CoinGecko users and allow them the opportunity to participate in the Raffle, you acknowledge and agree that any information you provide may be used by us in connection with the Raffle. To participate, users must follow these steps:
CoinGecko users will be able to redeem a NEAR NFT Raffle ticket with 800 candies on CoinGecko’s Candy Rewards Store between Thursday, May 18, 2023, 10pm EDT / Friday, May 19, 2023, 10am MYT through Sunday, May 21, 2023, 10pm EDT / Monday, May 22, 2023, 10am MYT.
Upon Raffle ticket redemption, users will receive a unique Candy Rewards code and a link to a Sweep Widget page. Users are required to fill in their details and may complete bonus actions like following CoinGecko and NEAR’s social accounts to boost chances of winning.
20,000 winners will be randomly selected to win a NEAR NFT each on Monday, May 22, 2023, of which 1 special winner* out of the 20,000 winners will additionally score a pair of tickets to NEARCON 2023 and $5,000 USD paid out in NEAR tokens. Winners will receive an email with a Keypom link allowing them to claim the NFT and open a NEAR Wallet in the week of May 22, 2023.
Winners will be required to hold the NFT in their NEAR wallet in order to be selected as the special winner that scores a pair of tickets to NEARCON 2023 and $5,000 USD worth of NEAR tokens.Only one winner will be selected as the special winner, of which will be notified via email and announced on the Near and CoinGecko’s social media channels on Friday, May 26, 2023.
Raffle Prize:
Selected winners will be announced and contacted via email. The Raffle prize consists of: 20,000 NFTs, 2x Tickets to NEARCON 2023 and $5,000 USD paid out in NEAR Tokens. The $5,000 amount can be used to book flights, accommodations, and as a meal stipend for NEARCON 2023.
5. Disclaimer:
The Prize is awarded ‘as is’ and without warranty of any kind, express or implied, including without limitation any warranty of merchantability or fitness for a particular purpose. The Raffle organizers and affiliated companies make no representations or warranties regarding the prize or its use and are not responsible for any claims arising out of or in connection with the prize or its use. Furthermore, we are not associated with any third-party companies or organizations mentioned in the raffle event (i.e. CoinGecko) or related promotional materials. The raffle event and prizes are solely sponsored and organized by us, and any reference to third-party companies or organizations is for informational purposes only.
Limitation of Liability:
The Raffle organizers, sponsors, and affiliated companies are not responsible for any injury, loss, or damage to property or person, including death, arising out of participation in the raffle or use or misuse of the prize.
Intellectual Property:
The Service, including all content provided by the expert(s) or other participants, is protected by copyright and other intellectual property laws. You may not reproduce, distribute, or create derivative works from any content provided by the Service without our prior written consent.
Modification of Terms:
We reserve the right to modify these Terms at any time without notice. Your continued use of the Service following any changes to these Terms constitutes your acceptance of the revised Terms.
Governing Law:
These Terms and your use of the Service are governed by the laws of Switzerland without regard to its conflict of laws provisions.
Dispute Resolution:
Any disputes arising out of or in connection with these Terms or the Service will be resolved through binding arbitration in accordance with the rules of the Switzerland Arbitration Association.
Entire Agreement:
These Terms constitute the entire agreement between you and us with respect to the Raffle and supersede all prior or contemporaneous agreements and understandings, whether written or oral.
Contact Information:
If you have any questions or comments about these Terms, please contact us at [email protected]. |
NEAR’s SF Blockchain Week Event Preview
COMMUNITY
October 5, 2018
Next week, the crypto community descends on San Francisco in force for the 2018 SF Blockchain Week. For the NEAR team, this is our home turf and a great chance to host our friends from around the world so we’ll be actively involved.
For those who can’t make it in person, our online panel event is listed as well.
The week is broken into three major parts:
The Ethereum SF Hackathon this weekend (Oct 5–7)
The Epicenter Conference on Monday-Tuesday (Oct 8–9)
The Crypto Economics Security Conference on Wednesday-Thursday (Oct 10–11).
We’ll be attending all of these events in different capacities, and here’s how you can meet the team or learn more about what we’re doing:
Friday Oct 5 – Sunday Oct 7: ETH SF
We love developing as much as you do so a number of NEAR team members will be participating in the ETH SF hackathon over the weekend. We won’t tell you our ideas yet… but we hope to see you there.
Tuesday Oct 9 @ 9am: (Talk) From Sharded Databases to Sharded Blockchains
NEAR CEO Alex Skidanov will be delivering a talk at the Epicenter Conference which dives into the details of what makes sharding difficult, how the lessons from the database world transfer to blockchain and why sharding on the state, in particular, is so important. The team will be on hand to answer questions.
You’ll need tickets to the conference for this but the video will be available afterward.
RSVP Here
Tuesday Oct 9 @ 3:30pm-5pm: (Hands-on Dev Workshop) Building & Deploying a Highly Scalable Smart Contract Game
NEAR Protocol powers a highly scalable, mobile-friendly decentralized app platform. In this workshop, NEAR Protocol’s co-founder Illia Polosukhin will walk the audience through the steps of building scalable smart contracts: from collectibles to interactive cross-contract game. The audience will then have a chance to compete in a competition to build the best AI smart contract for this game to win the most tokens.
Again, you’ll need tickets to the conference for this one.
RSVP Here
Tuesday Oct 9 @ 6:30pm-8:30pm: (Panel Discussion) ABC Spotlight: Sharding for Blockchain Scalability
This event is organized by ABC Blockchain Community, as part of the SF Blockchain Week and the Spotlight Program. Our spots are limited so RSVP today to secure your spot.
Join founders of Harmony, Quarkchain, MOAC and NEAR Protocol for a panel discussion on sharding including
How sharding can solve blockchain scalability
Mainstream sharding solutions and the differences between them
Security challenges of current sharding solutions
Sharding vs two-layer solution
How to build industry grade database sharding & apply it to the blockchain
RSVP Here
Wednesday Oct 10 @ 5:30pm-8:00pm: (Panel Discussion) 500 Blockchain: State of Scaling — Latest Progress & Challenges
NEAR joins Oasis, Solana and Celer Network on stage to discuss scalability in a panel moderated by Bonnie Cheung from 500 Startups.
Scalability is undoubtedly one of the key roadblocks facing the public blockchains today. Since late 2017, we have seen an explosion in the number of new projects and highly-experienced developers looking to solve the scalability challenge from all angles. As we approach Q4 of 2018, where are we in terms of development, breakthroughs, and challenges?
Join us for a fun evening of information sharing & networking over drinks and nibbles!
In this panel discussion, you will learn:
Latest developments & breakthroughs across scaling solutions
Current challenges they are working on
Key milestones for the next 6–12 months
How new developers, founders, industry experts can get involved
RSVP Here
Thursday Oct 11 @ 9:00am-10:00am: (Online Panel Discussion) Blockchain Sharding Approaches (with Ethereum Foundation)
Moderated by Ivan Bogatyy from Metastable Capital
This event will have three teams dedicated to Ethereum sharding joining the stage with the NEAR team. The topics to be discussed include:
The current state of sharding project development
Current challenges with the various sharding approaches
Key milestones and plans for next 6–12 months
Sharding questions asked by moderator
Sharding questions from the audience
The panel will include:
Alex Skidanov & Illia Polosukhin who work on sharding at NEAR
Justin Drake who works on sharding at Ethereum Foundation
Preston Van Loon who works on sharding at Prysmatic Labs
Mamy André-Ratsimbazafy & Ryan Lipscombe who work on sharding at Status.im / Nimbus
RSVP Here
Saturday Oct 13 @ 10:30am-12:30pm: (Talk) From Sharded Databases to Sharded Blockchains
In this workshop, NEAR Protocol co-founder Illia Polosukhin will walk the audience through…
1) What sharding is
2) Why sharding is important
3) What are the challenges with sharding
4) How to build and deploy a mobile-friendly game that runs on a sharded blockchain
The audience will then have a chance to take part in a competition to build the best AI smart contract for the game with a live scoreboard.
RSVP Here
https://upscri.be/633436/ |
---
title: What is the NEAR Governance Forum?
description: Your go-to place to discuss everything NEAR
---
## What is the NEAR Governance Forum?
The [NEAR Governance Forum](https://gov.near.org/) is the living hub of NEAR Ecosystem. Here, NEAR Community members, developers, and the NEAR Foundation team come together to enable ecosystem participants to build something great and expand the NEAR ecosystem.
Much like the NEAR blockchain itself, the forum is completely open, accessible, and transparent. We'd love to have you on board \(if you're not already\) to help build the NEARverse.
The forum is a great place to meet creative members of the community, and find out more about how they integrate NEAR into their projects.
There are active discussions about NEAR marketing, development, the various aspects of the NEAR ecosystem, and how to teach and/or learn about NEAR.
The form is also a great place to connect to the different regional hubs for NEAR, you can connect with the community in your region, and find NEAR community members from all over the world.
One last thing before you run off to [sign up](https://gov.near.org/), do check out the next page for the Forum Guidelines.
|
---
sidebar_position: 2
---
# BWE Overview
BOS Web Engine (BWE) is the next-gen decentralized frontend execution environment.
BWE brings decentralized frontend development significantly closer to standard React development and provides enhanced, browser-backed security.
Key Features:
- Upgrades developer experience (TypeScript, improved debugging)
- Access to more powerful components (npm packages, true hooks, standard JS environment)
- Performance optimization & tuning (configurable trust model)
- Increased security guarantees using iframe sandboxing, removing the burden of code-level security rules
:::note
"BOS Web Engine" is a working title and will change before launch
:::
## Example Component
`Foo.tsx`
```tsx
// import CSS module
import s from './Foo.module.css';
// import another BWE component
import Account from 'near://webengine.near/Account'
// import a BWE component from the same account using a relative path
import Post from './Post'
type Props = {
message?: string;
};
function Foo({ message = "Hello!" }: Props) {
return (
<div className={s.wrapper}>
<Account props={{accountId: 'bwe-demos.near'}} />
<Post props={{content: 'Hello World'}} />
<p>{message}</p>
</div>
);
}
export default Foo as BWEComponent<Props>;
```
`Foo.module.css`
```css
.wrapper {
display: flex;
flex-direction: column;
gap: 1rem;
}
```
## How it Works
1. Component code is executed with Preact in hidden iframes with sandboxing features enabled
2. When the component in the iframe has performed a render, it emits an event with the DOM produced
3. The outer window application (OWA) listens for render events from all iframes and stitches their DOM outputs together onto the visible page
Javascript runs in sandboxes, HTML/CSS is returned
 |
---
id: skeleton
title: Skeleton and Rust Architecture
sidebar_label: Contract Architecture
---
import {Github} from "@site/src/components/codetabs"
In this article, you'll learn about the basic architecture behind the NFT contract that you'll develop while following this _"Zero to Hero"_ series.
You'll discover the contract's layout and you'll see how the Rust files are structured in order to build a feature-complete smart contract.
:::info Skeleton Contract
You can find the skeleton contract in our [GitHub repository](https://github.com/near-examples/nft-tutorial/tree/main/nft-contract-skeleton)
:::
:::info New to Rust?
If you are new to Rust and want to dive into smart contract development, our [Quick-start guide](../../2.build/2.smart-contracts/quickstart.md) is a great place to start.
:::
---
## Introduction
This tutorial presents the code skeleton for the NFT smart contract and its file structure.
Once every file and functions have been covered, we will guide you through the process of building the mock-up contract to confirm that your Rust setup works.
---
## File structure
Following a regular [Rust](https://www.rust-lang.org/) project, the file structure for this smart contract has:
```
nft-contract
├── Cargo.lock
├── Cargo.toml
├── README.md
└── src
├── approval.rs
├── enumeration.rs
├── lib.rs
├── metadata.rs
├── mint.rs
├── nft_core.rs
├── events.rs
└── royalty.rs
```
- The file `Cargo.toml` defines the code dependencies
- The `src` folder contains all the Rust source files
<hr class="subsection" />
### Source files
Here is a brief description of what each source file is responsible for:
| File | Description |
|----------------------------------|---------------------------------------------------------------------------------|
| [approval.rs](#approvalrs) | Has the functions that controls the access and transfers of non-fungible tokens |
| [enumeration.rs](#enumerationrs) | Contains the methods to list NFT tokens and their owners |
| [lib.rs](#librs) | Holds the smart contract initialization functions |
| [metadata.rs](#metadatars) | Defines the token and metadata structure |
| [mint.rs](#mintrs) | Contains token minting logic |
| [nft_core.rs](#nft_corers) | Core logic that allows you to transfer NFTs between users. |
| [royalty.rs](#royaltyrs) | Contains payout-related functions |
| [events.rs](#events) | Contains events related structures |
:::tip
Explore the code in our [GitHub repository](https://github.com/near-examples/nft-tutorial/).
:::
---
## `approval.rs`
> This allows people to approve other accounts to transfer NFTs on their behalf.
This file contains the logic that complies with the standard's [approvals management](https://nomicon.io/Standards/Tokens/NonFungibleToken/ApprovalManagement) extension. Here is a breakdown of the methods and their functions:
| Method | Description |
|---------------------|-----------------------------------------------------------------------------------------------------------|
| **nft_approve** | Approves an account ID to transfer a token on your behalf. |
| **nft_is_approved** | Checks if the input account has access to approve the token ID. |
| **nft_revoke** | Revokes a specific account from transferring the token on your behalf. |
| **nft_revoke_all** | Revokes all accounts from transferring the token on your behalf. |
| **nft_on_approve** | This callback function, initiated during `nft_approve`, is a cross contract call to an external contract. |
<Github language="rust" start="4" end="33" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-basic/src/approval.rs" />
You'll learn more about these functions in the [approvals section](/tutorials/nfts/approvals) of the Zero to Hero series.
---
## `enumeration.rs`
> This file provides the functions needed to view information about NFTs, and follows the standard's [enumeration](https://nomicon.io/Standards/Tokens/NonFungibleToken/Enumeration) extension.
| Method | Description |
|--------------------------|------------------------------------------------------------------------------------|
| **nft_total_supply** | Returns the total amount of NFTs stored on the contract |
| **nft_tokens** | Returns a paginated list of NFTs stored on the contract regardless of their owner. |
| **nft_supply_for_owner** | Allows you view the total number of NFTs owned by any given user |
| **nft_tokens_for_owner** | Returns a paginated list of NFTs owned by any given user |
<Github language="rust" start="4" end="44" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-skeleton/src/enumeration.rs" />
You'll learn more about these functions in the [enumeration section](/tutorials/nfts/enumeration) of the tutorial series.
---
## `lib.rs`
> This file outlines what information the contract stores and keeps track of.
| Method | Description |
|----------------------|-------------------------------------------------------------------------------------------------|
| **new_default_meta** | Initializes the contract with default `metadata` so the user doesn't have to provide any input. |
| **new** | Initializes the contract with the user-provided `metadata`. |
:::info Keep in mind
The initialization functions (`new`, `new_default_meta`) can only be called once.
:::
<Github language="rust" start="47" end="73" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-skeleton/src/lib.rs" />
You'll learn more about these functions in the [minting section](/tutorials/nfts/minting) of the tutorial series.
---
## `metadata.rs`
> This file is used to keep track of the information to be stored for tokens, and metadata.
> In addition, you can define a function to view the contract's metadata which is part of the standard's [metadata](https://nomicon.io/Standards/Tokens/NonFungibleToken/Metadata) extension.
| Name | Description |
|-------------------|---------------------------------------------------------------------------------------------------------------|
| **TokenMetadata** | This structure defines the metadata that can be stored for each token (title, description, media, etc.). |
| **Token** | This structure outlines what information will be stored on the contract for each token. |
| **JsonToken** | When querying information about NFTs through view calls, the return information is stored in this JSON token. |
| **nft_metadata** | This function allows users to query for the contact's internal metadata. |
<Github language="rust" start="12" end="60" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-skeleton/src/metadata.rs" />
You'll learn more about these functions in the [minting section](/tutorials/nfts/minting) of the tutorial series.
---
## `mint.rs`
> Contains the logic to mint the non-fungible tokens
| Method | Description |
|--------------|-------------------------------------------|
| **nft_mint** | This function mints a non-fungible token. |
<Github language="rust" start="4" end="16" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-skeleton/src/mint.rs" />
---
## `nft_core.rs`
> Core logic that allows to transfer NFTs between users.
| Method | Description |
|--------------------------|-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| **nft_transfer** | Transfers an NFT to a receiver ID. |
| **nft_transfer_call** | Transfers an NFT to a receiver and calls a function on the receiver ID's contract. The function returns `true` if the token was transferred from the sender's account. |
| **nft_token** | Allows users to query for the information about a specific NFT. |
| **nft_on_transfer** | Called by other contracts when an NFT is transferred to your contract account via the `nft_transfer_call` method. It returns `true` if the token should be returned back to the sender. |
| **nft_resolve_transfer** | When you start the `nft_transfer_call` and transfer an NFT, the standard also calls a method on the receiver's contract. If the receiver needs you to return the NFT to the sender (as per the return value of the `nft_on_transfer` method), this function allows you to execute that logic. |
<Github language="rust" start="7" end="56" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-skeleton/src/nft_core.rs" />
You'll learn more about these functions in the [core section](/tutorials/nfts/core) of the tutorial series.
---
## `royalty.rs`
> Contains payout-related functions.
| Method | Description |
|-------------------------|---------------------------------------------------------------------------------------------------------------|
| **nft_payout** | This view method calculates the payout for a given token. |
| **nft_transfer_payout** | Transfers the token to the receiver ID and returns the payout object that should be paid for a given balance. |
<Github language="rust" start="3" end="17" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-skeleton/src/royalty.rs" />
You'll learn more about these functions in the [royalty section](/tutorials/nfts/royalty) of the tutorial series.
---
## `events.rs`
> Contains events-related structures.
| Method | Description |
|---------------------|-----------------------------------------------------|
| **EventLogVariant** | This enum represents the data type of the EventLog. |
| **EventLog** | Interface to capture data about an event. |
| **NftMintLog** | An event log to capture token minting. |
| **NftTransferLog** | An event log to capture token transfer. |
<Github language="rust" start="5" end="79" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-events/src/events.rs" />
You'll learn more about these functions in the [events section](/tutorials/nfts/events) of the tutorial series.
---
## Building the skeleton
If you haven't cloned the main repository yet, open a terminal and run:
```sh
git clone https://github.com/near-examples/nft-tutorial/
```
Next, go to the `nft-contract-skeleton/` folder and build the contract with `cargo-near`:
```sh
cd nft-tutorial
cd nft-contract-skeleton/
cargo near build
```
Since this source is just a skeleton you'll get many warnings about unused code, such as:
```
Compiling nft_contract_skeleton v0.1.0 (/Users/near-examples/Documents/my/projects/near/examples/nft-tutorial/nft-contract-basic)
│ warning: unused imports: `LazyOption`, `LookupMap`, `UnorderedMap`, `UnorderedSet`
│ --> src/lib.rs:3:29
│ |
│ 3 | use near_sdk::collections::{LazyOption, LookupMap, UnorderedMap, UnorderedSet};
│ | ^^^^^^^^^^ ^^^^^^^^^ ^^^^^^^^^^^^ ^^^^^^^^^^^^
│ |
│ = note: `#[warn(unused_imports)]` on by default
│
│ warning: unused import: `Base64VecU8`
│ --> src/lib.rs:4:28
│ |
│ 4 | use near_sdk::json_types::{Base64VecU8, U128};
│ |
│ warning: `nft_contract_skeleton` (lib) generated 48 warnings (run `cargo fix --lib -p nft_contract_skeleton` to apply 45 suggestions)
│ Finished release [optimized] target(s) in 11.01s
✓ Contract successfully built!
```
Don't worry about these warnings, you're not going to deploy this contract yet.
Building the skeleton is useful to validate that your Rust toolchain works properly and that you'll be able to compile improved versions of this NFT contract in the upcoming tutorials.
---
## Conclusion
You've seen the layout of this NFT smart contract, and how all the functions are laid out across the different source files.
Using `yarn`, you've been able to compile the contract, and you'll start fleshing out this skeleton in the next [Minting tutorial](/tutorials/nfts/minting).
:::note Versioning for this article
At the time of this writing, this example works with the following versions:
- rustc: `1.76.0`
- near-sdk-rs: `5.1.0`
- NFT standard: [NEP171](https://nomicon.io/Standards/Tokens/NonFungibleToken/Core), version `1.1.0`
:::
|
The Future of DAOs: Tooling, Standards, and Management
COMMUNITY
March 28, 2022
When DAOs, or decentralized autonomous organizations, entered the cultural lexicon in 2015, they were theoretical. No one had yet created one.
Fast forward to 2022 and they are, in many ways, the organizing principle of Web3. Decentralized and autonomous, with their own governance procedures and voting structures, DAOs are the entities that make Web3 go.
From 1 token-1 vote to delegated, role-based, and tiered token-based voting, they now come with a custom toolkit of roles, “access” levels, and more. And they’re only just starting to evolve.
“The DAO trend right now is about where NFTs were in 2020,” says Ozymandius, Co-founder and CPO of Open Forest Protocol (OFP). “Which means we are only starting to scratch the surface of customizations and functionality.”
But where are DAOs going as Web3 blockchain platforms, like NEAR, begin onboarding the masses? To get an idea of the future DAO landscape, the NEAR Foundation turned to a number of NEAR community members who are doing pioneering work with DAOs to find out.
Balancing creativity with coordination
To unleash the next wave of DAOs, Sputnik, Astro, and other NEAR-based launchpads will be vital.
The code that is Sputnik, the original DAO-creation machine, with its customization features was built with developers in mind. Sputnik allows for the funding of bounties on-chain, communication amongst DAOs, Council Structures for roles within a DAO (each with its own unique set of permissions), embedded Social $Tokens, on-chain polling of participants, and more. Sputnik’s feature set will only continue to grow in the future with the V3 release.
“Sputnik V2 is completely underrated with the level of customizations and permissions possible,” says Ozymandius. “From when Illia [Polosukhin, NEAR Protocol co-founder] initially presented it to a group of us in May of 2021, my first thought was: ‘Holy shit, you are going to be able to put the European Union on Sputnik V2’. I hope in next 6 months to a year that we start to see more sophisticated DAO customizations using Sputnik V2 and when Sputnik V3 comes out.”
Astro, on the other hand, is very plug-and-play—a more simplified DAO launchpad. OFP’s Ozymandias recommends Astro for those who want to creatively experiment with DAOs, which will help them flourish.
“More than anything, we need some creativity and R & D,” says Ozymandius. People who are looking to see what’s creatively possible instead of just making a product.
While great engines of creativity, DAOs can also be difficult to coordinate. To counter this, Ozymandius believes there will be more pooling of resources for coordinated purchases or acquisition, collective bonding of assets for treasury build-up, or collective pooling of assets for service offerings (LP DAOs, Data/Analytics DAOs), etc.
“I also think we are going to see them become more hierarchical to balance the most committed members versus the general community member,” Ozymandius says. “I also think we will see more care in the design of who can propose legislation to a DAO and vote on such legislation.”
Beyond the technical innovations, there is something more organic at work—more human. Tracey Bowen, Founder of H.E.R. DAO, a womxn-led developer DAO championing innovation and diversity, believes that in the future groups will probably be able to call themselves a DAO without ‘in-chain’ markers like a wallet or voting mechanisms.
“I see that shifting into people organizing themselves in a way which is more reflective of their actual goals,” says Bowen. “I’m actually in favor of relaxing the emphasis on automation and concentrating more on human expression in terms of organization being more fluid.”
In the last six months, DAOs have entered the cultural vernacular, with thought pieces appearing regularly in business publications like Forbes, Bloomberg, and others . More concretely, people have seen them far away from the Web3 world. In late 2021, ConstitutionDAO hit the headlines when it tried to collectively buy a copy of the U.S. Constitution.
As AstroDAO’s Jordan Gray notes, people are starting to understand the overall idea of DAOs, and the logistics of starting or participating in one. This curiosity will pave the way for the future of decentralized organizations.
“This opens up more questions that I don’t think anybody has answers to yet because there’s a lot of discovery happening,” says Gray. “That’s also the Web3 space in general: we are wayfinding. We have ideas on the ways these things can work, and we try them out. Sometimes it’s successful and we repeat. Sometimes it’s a failure and we course correct, figure out what went wrong, and how things could go better.”
Upgrades to DAO tooling & operations
DAOs will have more clear cut archetypes, with tools and systems to help run them. They will be legal entities, companies, and non-profits. They will also be one-off use cases, and often a type of community building, like a group of friends doing stuff together with a shared wallet.
“This would be accompanied by more clear legal precedents with regard to DAOs and trial-and-error-based learnings that set general guidelines for how they operate,” says Open Forest Protocol’s Rim Berjack.
Berjack, who has worked with DAOs for several years on a technical level, is keen on developing tooling, complimentary communication channels and platforms, and software or services for DAO treasury management.
“I’ve been noticing kernels of these tools emerging as we witness DAOs of great scale learn how to create formal systems and establish best practices, often evolving into a complex organism of hundreds of contributors,” she says. “I foresee the business of DAO tooling and management becoming an industry in itself as more projects that launch as DAOs or later become one bloom into multi-billion dollar enterprises.”
Chloe Lewis, Founder of Marma J Foundation, a Web3 platform for social good, also believes tooling will be vital. In general, tooling focused on treasury and asset management, or which gives DAOs legal frameworks to become fully incorporated entities. This will make Web3 more interactive with Web2 and the physical world.
“The difficulty lies mostly in the ‘legal wrapper’ that needs to be set up and the regulatory climate ambiguity,” Lewis says. “The Marma J Foundation is setting up a non-profit organization to support in this area, but it isn’t a process that can be done in a purely decentralized way. Once done, though, the foundation can interact with IRL aspects while the DAO interacts with Web3 aspects.”
Organizationally, DAOs could take any number of paths, and there won’t be a one-size-fits-all approach. Some DAOs might remain fiercely decentralized. Others could establish hierarchies, particularly those with large memberships.
“Realistically, I think we’ll see a spectrum of organizational structures depending on the character of the DAO,” says Berjack. “DAOs are a technology, a tool, and i’s a choice to implement familiar structures or entirely innovate new ways of decision making.”
For Illia Polosukhin, Co-founder of the NEAR Protocol, DAOs are a way to open up what he calls the “organization design space.”
“DAOs can program any kind of interaction between people, so we will see more and more new structures of people, compensation mechanics, and permissioned ways of interacting with an entity,” says Polosukhin. “At the same time, we will see more KYC (Know Your Customer), employment laws, and payroll integrations. This will bring existing DAOs closer to familiar company structures.”
“Decentralized doesn’t mean there is no need for coordination and leaders, and that needs to be more integrated into tooling and people’s interactions,” he says. “Making it clear who in the DAO is responsible for what direction of the work, who is contacting people, and how newcomers can onboard and become active members beyond just the onboarding guide in Discord.”
Multi-chain DAO standards
In Web3, each chain has its own approach, culture, tools, and this type of diversity is certainly a strength. But for a multi-chain Web3 future, DAO standards between blockchains will be necessary.
A working group called DAO Star is tackling this issue on NEAR. The goal is to normalize the way decentralized organizations publish information about their activities.
“It’s kind of like RSS for DAOs,” Astro’s Jorday Gray explains. “This is something that Deep DAO and Messari are part of as well. The ways DAOs transmit and publish information about themselves can show up in these aggregators, and it doesn’t matter what chain they’re on. I think that it’s essential to reach some standard point for these communications, so that everybody is interoperable.”
These standards will also be useful as more Web3 activities decentralize. With such standards and cross-chain bridges, DAOs will be able to work together in more seamless ways.
The multi-chain future will also see users participating in DAOs without a direct on-chain account where they function. They will also be able to trigger actions on other chains with tools like Rainbow Bridge.
“DAOs can have members represented by their addresses on other chains and read from the state of that chain, provided by Rainbow Bridge,” Polosukhin explains. “Similarly, a they can form a request to Rainbow Bridge to pass it to the other chain to execute it. This will provide a seamless way to onboard new users into DAOs independent of where the user is coming from.”
Marma J’s Lewis says interactions between DAOs are already quite frequent, albeit occasionally inefficient. “The agreements they have with each other will get much more complex and therefore potentially efficient and beneficial for the communities involved,” Lewis says.
Gray hopes to see core functionality, like aspects of the treasury and governance (voting), broken off from DAOs. Once this happens, then new, creative interfaces and experiences will be built around them, with which it and end users can interface.
“[Imagine] a musician or an artist that wants to up their engagement with a post on Twitter,” says Gray. “They could set up a smart contract that allows a user to link their wallet, link their Twitter account, and if they interact with the posts—retweet, like it, whatever—then they get something: either an NFT or fungible token or even some NEAR.”
And when an artist wants to spend 100 $NEAR on more engagement for new posts, they can put it to a vote in the DAO. Unlike Web2’s ad networks, Web3 would create a closer connection between fans and their favorite artists, musicians, and other creators. The layers between them won’t exist, and fans will be rewarded directly by the artist or DAO.
In the future, a constellation of interfaces could grow around and interact with a primary DAO, like the one envisioned above by Gray. For this to happen, though, the information on interfacing with them must defined more clearly. The problem is that smart contracts, like the ones behind DAOs, don’t really publish interfaces to themselves in a clean way.
“That’s something we’re doing currently with Astro DAO and TenK: for smart contracts to actually have some kind of manifesto about what they’re capable of doing,” Gray explains. “Once published as a JSON or some computer-readable format, then any React app or frontend could look at any smart contract and create an interface user interaction.”
“This will aid a ton with discoverability of contract feature sets, allowing for things like crawlers and searchable indices of smart contracts.”
When DAOs won’t be called DAOs
One day, DAOs will stop referring to themselves as DAOs. They will be, like their Web2 predecessors, just apps—autonomously running investment tools, community-focused organizations, sports teams, ReFi projects, and so much more. You name it and DAOs will be able to do it.
So, when the word “DAO” is no longer attached to an app or project’s name, it could be said that Web3 will have arrived in the mainstream consciousness.
“While the term helps distinguish it from centralized Web2 platforms, once mainstream culture fully grasps the DAO concept, the term will be rendered superfluous,” OFP’s Berjack says.
Polosukhin prefers the term “Digital Cooperative”, believing it gives people a better grasp of how DAOs can organize people in digital realms. “I think the more examples that are successful, the better intuition people will have around what they can do with them,” he says.
“Right now everything is very early and experimental,” adds Berjack. “So, more than anything, I am looking forward to the DAO’s conceptual maturity that will come with time.”
Stay tuned for the second installation of where DAOs will go back. In the meantime, read Part 1: How DAOs are Changing Everything, Part 2: Exploring Astro DAO, and Part 3: Life-Changing DAOs. |
---
id: upgrade-contract
title: Upgrading the Contract
sidebar_label: Upgrade a Contract
---
import {Github} from "@site/src/components/codetabs"
In this tutorial, you'll build off the work you previously did to implement the [minting functionality](/tutorials/nfts/minting) on a skeleton smart contract. You got to the point where NFTs could be minted and the wallet correctly picked up on the fact that you owned an NFT. However, it had no way of displaying the tokens since your contract didn't implement the method that the wallet was trying to call.
---
## Introduction
Today you'll learn about deploying patch fixes to smart contracts and you'll use that knowledge to implement the `nft_tokens_for_owner` function on the contract you deployed in the previous tutorial.
---
## Upgrading contracts overview {#upgrading-contracts}
Upgrading contracts, when done right, can be an immensely powerful tool. If done wrong, it can lead to a lot of headaches. It's important to distinguish between the code and state of a smart contract. When a contract is deployed on top of an existing contract, the only thing that changes is the code. The state will remain the same and that's where a lot of developer's issues come to fruition.
The NEAR Runtime will read the serialized state from disk and it will attempt to load it using the current contract code. When your code changes, it might not be able to figure out how to do this.
You need to strategically upgrade your contracts and make sure that the runtime will be able to read your current state with the new contract code. For more information about upgrading contracts and some best practices, see the NEAR SDK's [upgrading contracts](/sdk/rust/building/prototyping) write-up.
---
## Modifications to our contract {#modifications-to-contract}
In order for the wallet to properly display your NFTs, you need to implement the `nft_tokens_for_owner` method. This will allow anyone to query for a paginated list of NFTs owned by a given account ID.
To accomplish this, let's break it down into some smaller subtasks. First, you need to get access to a list of all token IDs owned by a user. This information can be found in the `tokens_per_owner` data structure. Now that you have a set of token IDs, you need to convert them into `JsonToken` objects as that's what you'll be returning from the function.
Luckily, you wrote a function `nft_token` which takes a token ID and returns a `JsonToken` in the `nft_core.rs` file. As you can guess, in order to get a list of `JsonToken` objects, you would need to iterate through the token IDs owned by the user and then convert each token ID into a `JsonToken` and store that in a list.
As for the pagination, Rust has some awesome functions for skipping to a starting index and taking the first `n` elements of an iterator.
Let's move over to the `enumeration.rs` file and implement that logic:
<Github language="rust" start="46" end="75" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-contract-basic/src/enumeration.rs" />
---
## Redeploying the contract {#redeploying-contract}
Now that you've implemented the necessary logic for `nft_tokens_for_owner`, it's time to build and re-deploy the contract to your account. Using the cargo-near, deploy the contract as you did in the previous tutorial:
```bash
cargo near deploy $NFT_CONTRACT_ID without-init-call network-config testnet sign-with-keychain send
```
Once the contract has been redeployed, let's test and see if the state migrated correctly by running a simple view function:
```bash
near view $NFT_CONTRACT_ID nft_metadata
```
This should return an output similar to the following:
```bash
{
spec: 'nft-1.0.0',
name: 'NFT Tutorial Contract',
symbol: 'GOTEAM',
icon: null,
base_uri: null,
reference: null,
reference_hash: null
}
```
**Go team!** At this point, you can now test and see if the new function you wrote works correctly. Let's query for the list of tokens that you own:
```bash
near view $NFT_CONTRACT_ID nft_tokens_for_owner '{"account_id": "'$NFT_CONTRACT_ID'", "limit": 5}'
```
<details>
<summary>Example response: </summary>
<p>
```bash
[
{
token_id: 'token-1',
owner_id: 'goteam.examples.testnet',
metadata: {
title: 'My Non Fungible Team Token',
description: 'The Team Most Certainly Goes :)',
media: 'https://bafybeiftczwrtyr3k7a2k4vutd3amkwsmaqyhrdzlhvpt33dyjivufqusq.ipfs.dweb.link/goteam-gif.gif',
media_hash: null,
copies: null,
issued_at: null,
expires_at: null,
starts_at: null,
updated_at: null,
extra: null,
reference: null,
reference_hash: null
}
}
]
```
</p>
</details>
---
## Viewing NFTs in the wallet {#viewing-nfts-in-wallet}
Now that your contract implements the necessary functions that the wallet uses to display NFTs, you should be able to see your tokens on display in the [collectibles tab](https://testnet.mynearwallet.com//?tab=collectibles).

---
## Conclusion
In this tutorial, you learned about the basics of [upgrading contracts](#upgrading-contracts). Then, you implemented the necessary [modifications to your smart contract](#modifications-to-contract) and [redeployed it](#redeploying-contract). Finally you navigated to the wallet collectibles tab and [viewed your NFTs](#viewing-nfts-in-wallet).
In the [next tutorial](/tutorials/nfts/enumeration), you'll implement the remaining functions needed to complete the [enumeration](https://nomicon.io/Standards/Tokens/NonFungibleToken/Enumeration) standard.
:::note Versioning for this article
At the time of this writing, this example works with the following versions:
- near-cli: `4.0.13`
- cargo-near `0.6.1`
- NFT standard: [NEP171](https://nomicon.io/Standards/Tokens/NonFungibleToken/Core), version `1.1.0`
:::
|
How Solaire is Revolutionizing the Fashion Industry with Web3 Technology and Building the Retail Infrastructure on NEAR
CASE STUDIES
March 30, 2023
Solaire – an exciting web3 retail platform that merges physical and digital assets – is revolutionizing the way consumers interact with fashion brands. By harnessing the power of web3 and the NEAR blockchain, Solaire is building out key protocol infrastructure and APIs that will expand decentralized retail and unlock new opportunities for both shoppers and fashion brands alike.
Solaire is pioneering phygital use cases and brands will be able to leverage the NEAR blockchain in tandem with Solair’s powerful APIs to engage with their audience in novel ways. Solaire’s suite of APIs seamlessly integrates with a brand’s existing retail channels. This enables new revenue channels and richer data.
Solaire’s entire backend is fully on-chain and facilitates an easy-to-use web2 interface, with no wallet or crypto needed from shoppers. The NEAR blockchain helps Solaire provide these benefits for brands, as well as unique integrations with NEAR. This includes Solaire integration with the NEAR Mainnet, native NEAR wallet support, and the NEAR token as a payment option.
How Solaire is Changing Fashion with Phygital
Solaire establishes a 1:1 connection between a physical object and its digital twin. Brands can then leverage Solaire’s infrastructure and suite of APIs to engage customers with NFTs and phygital experiences in a seamless, user-friendly way, removing some of the typical web3 friction points.
“We aim to do all the hard work on the backend so that brands and consumers can enjoy a familiar user experience with all the benefits of Web3 but without the hassle of dealing with wallet or crypto,” says Vivian Zhang, CEO of Solaire. “And NEAR’s blockchain is perfectly suited to run enterprise-level protocols to ensure a seamless and frictionless user experience.”
Soliare infrastructure helps brands create meaningful digital twins for physical goods, as well as providing a means for consumers to buy and trade phygital assets. Solaire allows for minting unique NFTs attached to physical goods and offers an end-to-end solution for securing and tracking a product from manufacturing through its entire product lifecycle.
Solaire has already collaborated with major fashion brands like Freeman’s Limited Edition Phygital Harry Potter™ Collection and Ilona Song. Smart contracts facilitate shipping, returns, and exchanges all on the NEAR blockchain This allows customers to shop and purchase with transparency and confidence.
Benefits of Phygital Assets for Fashion Brands
Phygital assets are a new way for fashion brands to engage consumers, combining the physical and digital worlds to create a more immersive shopping experience. For example, when a customer buys a dress in real life, they also receive a digital wearable. This can be in the form of an AR filter, a digital skin in their favorite game, or to wear in the metaverse.
“Solaire’s mission is to empower the retail industry with web3 tools to cultivate a more connected and automated ecosystem,” Zhang continues. “With our suite of APIs, brands are able to maximize revenue and gain valuable data from digital assets, facilitate the secondary market, and build community.”
Brands can then use Solaire as a powerful CRM tool. Through the digital twin in customers’ wallets, brands will have a constant open channel of communication with both the primary customer and secondary owners. Smart contracts also automate royalties from secondary selling, giving brands visibility into trading data.
Another exciting feature is the secondary market experience brands can now provide. Solaire’s backend enables peer-to-peer physical asset trading without a middleman, utilizing blockchain-secured data and smart contracts. Buyers and sellers can independently confirm possession and authenticity of assets on-chain, and smart contracts resolve any disputes.
Solaire and NEAR Building a Phygital Fashion Future
“We’re building on NEAR because it champions community, and it’s one we have found to be incredibly supportive,” remarks Vivian Zhang, CEO of Solaire. “We’ve been able to connect and collaborate with so many partners as we build an entire retail ecosystem together on NEAR.”
As fashion brands recognize the value of web3, NFTs, and phygital technology as important audience touchpoints, Solaire has the potential to partner with even more fashion brands and designers. Solaire’s game-changing approach and tech stack – aided by the NEAR blockchain – will bring web3 to more fashionistas without some of the usual pain points.
“Together with NEAR, we hope to lay out the Web3 infrastructure for the retail industry to onboard the next billion users into web3,” concluded Zhang. |
---
id: skeleton
title: Skeleton and Rust Architecture
sidebar_label: Contract Architecture
---
import {Github} from "@site/src/components/codetabs"
> In this article, you'll learn about the basic architecture behind the FT contract that you'll develop while following this _"Zero to Hero"_ series.
> You'll discover the contract's layout and you'll see how the Rust files are structured in order to build a feature-complete smart contract.
:::info New to Rust?
If you are new to Rust and want to dive into smart contract development, our [Quick-start guide](../../2.build/2.smart-contracts/quickstart.md) is a great place to start.
:::
---
## Introduction
This tutorial presents the code skeleton for the FT smart contract and its file structure.
You'll find how all the functions are laid out as well as the missing Rust code that needs to be filled in.
Once every file and function has been covered, you'll go through the process of building the mock-up contract to confirm that your Rust toolchain works as expected.
## File structure
The repository comes with many different folders. Each folder represents a different milestone of this tutorial starting with the skeleton folder and ending with the finished contract folder. If you step into any of these folders, you'll find that they each follow a regular [Rust](https://www.rust-lang.org/) project. The file structure for these smart contracts have:
- `Cargo.toml` file to define the code dependencies (similar to `package.json` in JavaScript and node projects)
- `src` folder where all the Rust source files are stored
- `target` folder where the compiled `wasm` will output to
- `build.sh` script that has been added to provide a convenient way to compile the source code
### Source files
| File | Description |
| -------------------------------- | -------------------------------------------------------------------------------- |
| [ft_core.rs](#corers) | Contains the logic for transferring and controlling FTs. This file represents the implementation of the [core](https://nomicon.io/Standards/Tokens/FungibleToken/Core) standard. | |
| [lib.rs](#librs) | Holds the smart contract initialization functions and dictates what information is kept on-chain. |
| [metadata.rs](#metadatars) | Defines the metadata structure. This file represents the implementation of the [metadata](https://nomicon.io/Standards/Tokens/FungibleToken/Metadata) extension of the standard. |
| [storage.rs](#storagers) | Contains the logic for registration and storage. This file represents the implementation of the [storage management](https://nomicon.io/Standards/StorageManagement) standard. |
```
skeleton
├── Cargo.lock
├── Cargo.toml
├── build.sh
└── src
├── ft_core.rs
├── lib.rs
├── metadata.rs
└── storage.rs
```
:::tip
Explore the code in our [GitHub repository](https://github.com/near-examples/ft-tutorial/tree/main/1.skeleton).
:::
---
## `ft_core.rs`
> Core logic that allows you to transfer FTs between users and query for important information.
| Method | Description |
| ------------------------ | --------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| **ft_transfer** | Transfers a specified amount of FTs to a receiver ID.|
| **ft_transfer_call** | Transfers a specified amount of FTs to a receiver and attempts to perform a cross-contract call on the receiver’s contract to execute the `ft_on_transfer` method. The implementation of this `ft_on_transfer` method is up to the contract writer. You’ll see an example implementation in the marketplace section of this tutorial. Once `ft_on_transfer` finishes executing, `ft_resolve_transfer` is called to check if things were successful or not.|
| **ft_total_supply** | Returns the total amount of fungible tokens in circulation on the contract. |
| **ft_balance_of** | Returns how many fungible tokens a specific user owns.|
| **ft_on_transfer** | Method that lives on a receiver's contract. It is called when FTs are transferred to the receiver's contract account via the `ft_transfer_call` method. It returns how many FTs should be refunded back to the sender. |
| **ft_resolve_transfer** | Invoked after the `ft_on_transfer` is finished executing. This function will refund any FTs not used by the receiver contract and will return the net number of FTs sent to the receiver after the refund (if any). |
<Github language="rust" start="61" end="166" url="https://github.com/near-examples/ft-tutorial/blob/main/1.skeleton/src/ft_core.rs" />
You'll learn more about these functions in the [circulating supply](/tutorials/fts/circulating-supply) and [transfers](/tutorials/fts/transfers) sections of the tutorial series.
---
## `lib.rs`
> This file outlines what information the contract stores and keeps track of.
| Method | Description |
| -------------------- | ----------------------------------------------------------------------------------------------- |
| **new_default_meta** | Initializes the contract with default `metadata` so the user doesn't have to provide any input. In addition, a total supply is passed in which is sent to the owner |
| **new** | Initializes the contract with the user-provided `metadata` and total supply. |
:::info Keep in mind
The initialization functions (`new`, `new_default_meta`) can only be called once.
:::
<Github language="rust" start="34" end="58" url="https://github.com/near-examples/ft-tutorial/blob/main/1.skeleton/src/lib.rs" />
You'll learn more about these functions in the [define a token](2-define-a-token.md) section of the tutorial series.
---
## `metadata.rs`
> This file is used to outline the metadata for the Fungible Token itself.
> In addition, you can define a function to view the contract's metadata which is part of the standard's [metadata](https://nomicon.io/Standards/Tokens/FungibleToken/Metadata) extension.
| Name | Description |
| ----------------- | ------------------------------------------------------------------------------------------------------------- |
| **FungibleTokenMetadata** | This structure defines the metadata for the fungible token. |
| **ft_metadata** | This function allows users to query for the token's metadata |
<Github language="rust" start="10" end="30" url="https://github.com/near-examples/ft-tutorial/blob/main/1.skeleton/src/metadata.rs" />
You'll learn more about these functions in the [define a token](2-define-a-token.md) section of the tutorial series.
---
## `storage.rs`
> Contains the registration logic as per the [storage management](https://nomicon.io/Standards/StorageManagement) standard.
| Method | Description |
| ----------------------- | ------------------------------------------------------------------------------------------------------------- |
| **storage_deposit** | Payable method that receives an attached deposit of Ⓝ for a given account. This will register the user on the contract. |
| **storage_balance_bounds** | Returns the minimum and maximum allowed storage deposit required to interact with the contract. In the FT contract's case, min = max.|
| **storage_balance_of** | Returns the total and available storage paid by a given user. In the FT contract's case, available is always 0 since it's used by the contract for registration and you can't overpay for storage. |
<Github language="rust" start="79" end="106" url="https://github.com/near-examples/ft-tutorial/blob/main/1.skeleton/src/storage.rs" />
:::tip
You'll learn more about these functions in the [storage](4.storage.md) section of the tutorial series.
:::
## Building the skeleton
- If you haven't cloned the main repository yet, open a terminal and run:
```sh
git clone https://github.com/near-examples/ft-tutorial/
```
- Next, build the skeleton contract with the build script found in the `1.skeleton/build.sh` file.
```sh
cd ft-tutorial/1.skeleton
./build.sh
cd ..
```
Since this source is just a skeleton you'll get many warnings about unused code, such as:
```
= note: `#[warn(dead_code)]` on by default
warning: constant is never used: `GAS_FOR_RESOLVE_TRANSFER`
--> src/ft_core.rs:5:1
|
5 | const GAS_FOR_RESOLVE_TRANSFER: Gas = Gas(5_000_000_000_000);
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
warning: constant is never used: `GAS_FOR_FT_TRANSFER_CALL`
--> src/ft_core.rs:6:1
|
6 | const GAS_FOR_FT_TRANSFER_CALL: Gas = Gas(25_000_000_000_000 + GAS_FOR_RESOLVE_TRANSFER.0);
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
warning: `fungible-token` (lib) generated 25 warnings
Finished release [optimized] target(s) in 1.93s
✨ Done in 2.03s.
```
Don't worry about these warnings, you're not going to deploy this contract yet.
Building the skeleton is useful to validate that your Rust toolchain works properly and that you'll be able to compile improved versions of this FT contract in the upcoming tutorials.
---
## Conclusion
You've seen the layout of this FT smart contract, and how all the functions are laid out across the different source files.
Using `yarn`, you've been able to compile the contract, and you'll start fleshing out this skeleton in the next [section](/tutorials/fts/circulating-supply) of the tutorial.
:::note Versioning for this article
At the time of this writing, this example works with the following versions:
- rustc: `1.6.0`
- near-sdk-rs: `4.0.0`
:::
|
---
id: faq
title: FAQ for NEAR JavaScript API
sidebar_label: FAQ
---
A collection of Frequently Asked Questions by the community.
## General {#general}
### Can I use `near-api-js` on a static html page? {#can-i-use-near-api-js-on-a-static-html-page}
You can load the script from a CDN.
```html
<script src="https://cdn.jsdelivr.net/npm/near-api-js@0.45.1/dist/near-api-js.min.js"></script>
```
:::note
Make sure you load the latest version.
Versions list is on [npmjs.com](https://www.npmjs.com/package/near-api-js)
:::
<details>
<summary>Example Implementation</summary>
<p>
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<ul id="messages"></ul>
<textarea id="text" placeholder="Add Message"></textarea>
<button id="add-text">Add Text</button>
<script src="https://cdn.jsdelivr.net/npm/near-api-js@0.45.1/dist/near-api-js.min.js"></script>
<script>
// connect to NEAR
const near = new nearApi.Near({
keyStore: new nearApi.keyStores.BrowserLocalStorageKeyStore(),
networkId: 'testnet',
nodeUrl: 'https://rpc.testnet.near.org',
walletUrl: 'https://testnet.mynearwallet.com/'
});
// connect to the NEAR Wallet
const wallet = new nearApi.WalletConnection(near, 'my-app');
// connect to a NEAR smart contract
const contract = new nearApi.Contract(wallet.account(), 'guest-book.testnet', {
viewMethods: ['getMessages'],
changeMethods: ['addMessage']
});
const button = document.getElementById('add-text');
if (!wallet.isSignedIn()) {
button.textContent = 'SignIn with NEAR'
}
// call the getMessages view method
contract.getMessages()
.then(messages => {
const ul = document.getElementById('messages');
messages.forEach(message => {
const li = document.createElement('li');
li.textContent = `${message.sender} - ${message.text}`;
ul.appendChild(li);
})
});
// Either sign in or call the addMessage change method on button click
document.getElementById('add-text').addEventListener('click', () => {
if (wallet.isSignedIn()) {
contract.addMessage({
args: { text: document.getElementById('text').value },
amount: nearApi.utils.format.parseNearAmount('1')
})
} else {
wallet.requestSignIn({
contractId: 'guest-book.testnet',
methodNames: ['getMessages', 'addMessage']
});
}
});
</script>
</body>
</html>
```
</p>
</details>
---
### What front-end frameworks can I use the JavaScript API with?
The JavaScript API is framework-agnostic. You can include it in any front-end framework, such as React, Vue, Angular, and others.
You can use [`create-near-app`](https://github.com/near/create-near-app) to quickly bootstrap projects with different templates:
npx create-near-app
### Can I use the JavaScript API with mobile JavaScript frameworks such as React Native?
The JavaScript API can be used in most JavaScript runtimes, and under the hood, it’s an abstraction over NEAR’s [RPC API](/api/rpc/introduction). However, notice that the Wallet can’t be used everywhere. For example, in React Native apps you’ll be able to use the Wallet in web versions of the apps, but it won’t work in the native app deployments.
You can use the Wallet in `WebView` components in iOS or Android, however be aware that it uses `LocalStorage` for `KeyStore`, and it’s your responsibility to persist the storage when you manage loading of `WebView` components.
---
## Transactions {#transactions}
### How to check the status of transaction
Please refer to examples about transactions in the [Cookbook](/tools/near-api-js/cookbook).
### How transactions are signed and sent by near-api-js
There are a few steps involved before transaction data is communicated to the network and eventually included in a block. The following steps are taken when creating, signing and ultimately a transaction from a user's account:
1. The user creates a transaction object using the [`account.signAndSendTransaction` method](https://github.com/near/near-api-js/blob/f78616480ba84c73f681211fe6266bd2ed2b9da1/packages/near-api-js/src/account.ts#L200). This method accepts an array of actions and returns an object for the outcome of the transaction.
2. The transaction is signed using the [`account.signTransaction` method](https://github.com/near/near-api-js/blob/f78616480ba84c73f681211fe6266bd2ed2b9da1/packages/near-api-js/src/account.ts#L204). This method accepts an array of actions and returns a signed transaction object.
3. The signed transaction object is sent to the network using the [`account.connection.provider.sendTransaction` method](https://github.com/near/near-api-js/blob/f78616480ba84c73f681211fe6266bd2ed2b9da1/packages/near-api-js/src/account.ts#L208). This method accepts a signed transaction object and returns a transaction hash. This step [performs the borsh serialization of the transaction object](https://github.com/near/near-api-js/blob/f78616480ba84c73f681211fe6266bd2ed2b9da1/packages/near-api-js/src/providers/json-rpc-provider.ts#L80) and calls the [`broadcast_tx_commit` JSON RPC method with the serialized transaction object encoded in base64](https://github.com/near/near-api-js/blob/f78616480ba84c73f681211fe6266bd2ed2b9da1/packages/near-api-js/src/providers/json-rpc-provider.ts#L81).
### How to send batch transactions
You may batch send transactions by using the `signAndSendTransaction({})` method from `account`. This method takes an array of transaction actions, and if one fails, the entire operation will fail. Here's a simple example:
```js
const { connect, transactions, keyStores } = require("near-api-js");
const fs = require("fs");
const path = require("path");
const homedir = require("os").homedir();
const CREDENTIALS_DIR = ".near-credentials";
const CONTRACT_NAME = "spf.idea404.testnet";
const WASM_PATH = path.join(__dirname, "../build/uninitialized_nft.wasm");
const credentialsPath = path.join(homedir, CREDENTIALS_DIR);
const keyStore = new keyStores.UnencryptedFileSystemKeyStore(credentialsPath);
const config = {
keyStore,
networkId: "testnet",
nodeUrl: "https://rpc.testnet.near.org",
};
sendTransactions();
async function sendTransactions() {
const near = await connect({ ...config, keyStore });
const account = await near.account(CONTRACT_NAME);
const args = { some_field: 1, another_field: "hello" };
const balanceBefore = await account.getAccountBalance();
console.log("Balance before:", balanceBefore);
try {
const result = await account.signAndSendTransaction({
receiverId: CONTRACT_NAME,
actions: [
transactions.deployContract(fs.readFileSync(WASM_PATH)), // Contract does not get deployed
transactions.functionCall("new", Buffer.from(JSON.stringify(args)), 10000000000000, "0"), // this call fails
transactions.transfer("1" + "0".repeat(24)), // 1 NEAR is not transferred either
],
});
console.log(result);
} catch (e) {
console.log("Error:", e);
}
const balanceAfter = await account.getAccountBalance();
console.log("Balance after:", balanceAfter);
}
```
```
Balance before: {
total: '49987878054959838200000000',
stateStaked: '4555390000000000000000000',
staked: '0',
available: '45432488054959838200000000'
}
Receipts: 2PPueY6gnA4YmmQUzc8DytNBp4PUpgTDhmEjRSHHVHBd, 3isLCW9SBH1MrPjeEPAmG9saHLj9Z2g7HxzfBdHmaSaG
Failure [spf.idea404.testnet]: Error: {"index":1,"kind":{"ExecutionError":"Smart contract panicked: panicked at 'Failed to deserialize input from JSON.: Error(\"missing field `owner_id`\", line: 1, column: 40)', nft/src/lib.rs:47:1"}}
Error: ServerTransactionError: {"index":1,"kind":{"ExecutionError":"Smart contract panicked: panicked at 'Failed to deserialize input from JSON.: Error(\"missing field `owner_id`\", line: 1, column: 40)', nft/src/lib.rs:47:1"}}
at parseResultError (/Users/dennis/Code/naj-test/node_modules/near-api-js/lib/utils/rpc_errors.js:31:29)
at Account.<anonymous> (/Users/dennis/Code/naj-test/node_modules/near-api-js/lib/account.js:156:61)
at Generator.next (<anonymous>)
at fulfilled (/Users/dennis/Code/naj-test/node_modules/near-api-js/lib/account.js:5:58)
at processTicksAndRejections (node:internal/process/task_queues:96:5) {
type: 'FunctionCallError',
context: undefined,
index: 1,
kind: {
ExecutionError: 'Smart contract panicked: panicked at \'Failed to deserialize input from JSON.: Error("missing field `owner_id`", line: 1, column: 40)\', nft/src/lib.rs:47:1'
},
transaction_outcome: {
block_hash: '5SUhYcXjXR1svCxL5BhCuw88XNdEjKXqWgA9X4XZW1dW',
id: 'SKQqAgnSN27fyHpncaX3fCUxWknBrMtxxytWLRDQfT3',
outcome: {
executor_id: 'spf.idea404.testnet',
gas_burnt: 4839199843770,
logs: [],
metadata: [Object],
receipt_ids: [Array],
status: [Object],
tokens_burnt: '483919984377000000000'
},
proof: [ [Object], [Object], [Object], [Object], [Object] ]
}
}
Balance after: {
total: '49985119959346682700000000',
stateStaked: '4555390000000000000000000',
staked: '0',
available: '45429729959346682700000000'
}
```
You may also find an example of batch transactions in the [Cookbook](/tools/near-api-js/cookbook).
---
## Accounts {#accounts}
### What’s the difference between `Account` and `ConnectedWalletAccount`?
Interaction with the wallet is only possible in a web-browser environment because NEAR’s Wallet is web-based.
The difference between `Account` and `ConnectedWalletAccount` is mostly about the way it signs transactions. The `ConnectedWalletAccount` uses the wallet to approve transactions.
Under the hood the `ConnectedWalletAccount` inherits and overrides some methods of `Account`.
### How to create implicit accounts?
You can read about it in the article about [Implicit Accounts](https://docs.near.org/integrations/implicit-accounts).
---
## Contracts {#contracts}
### How do I attach gas / a deposit? {#how-do-i-attach-gas--a-deposit}
After [contract is instantiated](/tools/near-api-js/quick-reference#load-contract) you can then call the contract and specify the amount of attached gas.
```js
await contract.method_name(
{
arg_name: "value", // argument name and value - pass empty object if no args required
},
"300000000000000", // attached GAS (optional)
"1000000000000000000000000" // attached deposit in yoctoNEAR (optional)
);
```
---
## Common Errors {#common-errors}
### RPC Errors
Refer to the exhaustive [list of error messages](https://github.com/near/near-api-js/blob/16ba17251ff7d9c8454261001cd6b87e9a994789/packages/near-api-js/src/res/error_messages.json)
that RPC endpoints throws and JavaScript API propagates.
### Missing contract methods {#missing-contract-method}
When constructing a `Contract` instance on the client-side, you need to specify
the contract's methods. If you misspell, mismatch, or miss method names - you'll
receive errors about missing methods.
There are a few cases of missing or wrong methods:
- When you call a method you didn't specify in the constructor.
- When you call a method that doesn't exist on the blockchain's contract (but you did specify it in the client-side constructor).
- When you mismatch between `viewMethods` and `changeMethods`.
For example, let's look at the following contract code.
It contains one `view` and one `call` method:
```js
@NearBindgen
class MyContract extends NearContract {
constructor() { super(); }
@view
method_A_view(): string {
return 'Hi';
}
@call
method_B_call(): void {}
}
```
#### Client-side errors for missing methods
##### `TypeError: contract.METHOD_NAME is not a function`
The following contract constructor declares only `method_A_view`, it doesn't declare `method_B_call`
```js
const contract = await new nearAPI.Contract(
walletConnection.account(), 'guest-book.testnet',
{
viewMethods: ['method_A_view'], // <=== Notice this
changeMethods: [], // <=== Notice this
sender: walletConnection.getAccountId(),
}
);
// This will work because we declared `method_A_view` in constructor
await contract.method_A_view();
// This will throw `TypeError: contract.method_B_call is not a function`
// because we didn't declare `method_B_call` in constructor,
// even if it exists in the real contract.
await contract.method_B_call();
// This will also throw `TypeError: contract.method_C is not a function`,
// not because `method_C` doesn't exist on the contract, but because we didn't declare it
// in the client-side constructor.
await contract.method_C();
```
#### RPC errors for missing methods
##### `wasm execution failed with error: FunctionCallError(MethodResolveError(MethodNotFound))`
In this example we specify and call a method, but this method doesn't exist on the blockchain:
```js
const contract = await new nearAPI.Contract(
// ...
{
viewMethods: ["method_C"], // <=== method_C doesn't exist on the contract above
changeMethods: [],
// ...
}
);
// We did specify `method_C` name in constructor, so this function exists on client-side `contract` instance,
// but a method with this name does not exist on the actual contract on the blockchain.
// This will return an error from RPC call `FunctionCallError(MethodResolveError(MethodNotFound))`
// and will throw it on the client-side
await contract.method_C();
// Notice: if we call `method_A_view` we get `TypeError: contract.method_A_view is not a function`.
// Even though the method exists on the actual blockchain contract,
// we didn't specify `method_A_view` in the contract's client-side constructor.
await contract.method_A_view();
```
##### `wasm execution failed with error: FunctionCallError(HostError(ProhibitedInView { method_name: "storage_write" }))`
Last case is when you mismatch `viewMethods` and `changeMethods`.
In the contract above we declared:
- A `@view` method named `method_A_view`
- A `@call` method named `method_B_call`
In a client-side constructor, the contract's `@view` method names must be specified under `viewMethods`,
and the contract's `@call` method names must be specified under `changeMethods`.
If we mismatch between the types we will receive errors.
For example:
```js
const contract = await new nearAPI.Contract(
// ...
{
viewMethods: ['method_B_call'], // <=== here should be `method_A_view`
changeMethods: ['method_A_view'], // <=== and here should be `method_B_call`
// ...
}
);
// This will return an error from RPC call and throw:
// `wasm execution failed with error: FunctionCallError(HostError(ProhibitedInView { method_name: "storage_write" }))`
// This error indicates that we are trying to call a state-changing method but declare it as a read-only method in client-side.
await contract.method_B_call();
// The following behavior is undefined and might not work as expected.
// `method_A_veiw` should be declared under `viewMethods` and in our example here we declare it under `changeMethods`.
await contract.method_A_view();
```
### Class `{X}` is missing in schema: publicKey
There is currently a known issue with the JavaScript API library, when you `import` it more than once
it might cause a namespace collision.
A temporary workaround: make sure you don't re-import it, for example when running tests.
---
### `regeneratorRuntime` is not defined {#regeneratorruntime-is-not-defined}
You are probably using [Parcel](https://parceljs.org/) like we do in [other examples](https://github.com/near-examples). Please make sure you have this line at the top of your main JS file. (Most likely `index.js`):
```js
import "regenerator-runtime/runtime";
```
- Also, ensure the dependencies for this are added to the project by checking the dev dependencies in your `package.json`. If not found you can install this by running the following in your terminal:
```bash
npm install regenerator-runtime --save-dev
```
---
### Window error using `Node.js` {#window-error-using-nodejs}
You're maybe using a KeyStore that's for the browser. Instead, use a [filesystem key](/tools/near-api-js/quick-reference#key-store) or private key string.
**Browser KeyStore:**
```js
const { keyStores } = require("near-api-js");
const keyStore = new keyStores.BrowserLocalStorageKeyStore();
```
**FileSystem KeyStore:**
```js
const { keyStores } = require("near-api-js");
const KEY_PATH = "~./near-credentials/testnet/example-account.json";
const keyStore = new keyStores.UnencryptedFileSystemKeyStore(KEY_PATH);
```
|
---
id: faq
title: Integrator FAQ
sidebar_label: Integrator FAQ
---
## Orientation
### What is a good project summary for NEAR?
NEAR is a sharded, public, proof-of-stake blockchain and smart contract platform. It is built in Rust and contracts compile to WASM. It is conceptually similar to Ethereum 2.0.
### What's special about NEAR?
NEAR is the blockchain for builders.
If you understand the basics of web development, you can write, test and deploy scalable decentralized applications in minutes on the most developer-friendly blockchain without having to learn new tools or languages.
### Is NEAR open source?
Yes. Have a look at our [GitHub organization](https://github.com/near).
### How are cryptographic functions used?
We support both `secp256k1` and `ed25519` for account keys and `ed25519` for signing transactions. We currently use the `ed25519_dalek` and `sha2` libraries for crypto.
### Do you have any on-chain governance mechanisms?
NEAR does not have any on-chain governance at the moment. Any changes to state or state transition function must be done through a hard fork.
### Do you have a bug-bounty program?
Our plan is to have a transparent Bug Bounty program with clear guidelines for paying out to those reporting issues. Payments will likely be based on publicly available rankings provided by protocol developers based on issue severity.
### What contracts should we be aware of right now?
We have developed a number of [initial contracts](https://github.com/near/core-contracts) with **ones in bold** being most mature at time of writing
- **Staking Pool / Delegation contract**
- **Lockup / Vesting contract**
- Whitelist Contract
- Staking Pool Factory
- Multisig contract
### Do you have a cold wallet implementation (ie. Ledger)?
https://github.com/near/near-ledger-app
## Validators
### What is the process for becoming a validator?
Validation is permissionless and determined via auction. Parties who want to become a validator submit a special transaction to the chain one day ahead which indicates how many tokens they want to stake. An auction is run which determines the minimum necessary stake to get a validation seat during the next epoch and, if the amount submitted is greater than the minimum threshold, the submitter will validate at least one shard during the next epoch.
### How long does a validator remain a validator?
A validator will stop being a validator for the following reasons:
- Not producing enough blocks or chunks.
- Not getting elected in the auction for next epoch because their stake is not large enough.
- Getting slashed.
Otherwise a validator will remain a validator indefinitely.
Validator election happens in epochs. The [Nightshade whitepaper](/docs/Nightshade.pdf) introduces epochs this way: "the maintenance of the network is done in epochs" where an epoch is a period of time on the order of half a day.
At the beginning of each epoch, some computation produces a list of validators for the _very next epoch_.
The input to this computation includes all accounts that have "raised their hand to be a validator"
by submitting a special transaction ([`StakeAction`](https://nomicon.io/RuntimeSpec/Actions.html#stakeaction))
expressing the commitment of some amount of tokens over the system's staking threshold,
as well as validators from the previous epoch.
The output of this computation is a list of the validators for the very next epoch.
### What is the penalty for misbehaving validators?
Validators are not slashed for being offline but they do miss out on the rewards of validating. Validators who miss too many blocks or chunks will be removed from the validation set in the next auction and not get any reward (but, again, without slashing).
Any foul play on the part of the validator that is detected by the system may result in a "slashing event" where the validator is marked as out of integrity and forfeit their stake (according to some formula of progressive severity). The slashed stake is burnt.
### What is the mechanism for delegating stake to validators?
NEAR supports separate validation keys that can be used in smart contracts to delegate stake. Delegation is done via smart contract which allows for a validator to define a custom way to collect stake, manage it and split rewards. This also allows validators to provide leverage or derivatives on stake. Delegated stake will be slashed like any other stake if the node misbehaves.
If a validator misbehaves the funds of the delegators are also slashed. There is no waiting period for delegators to withdraw their stake.
### Does a validator control funds that have been delegated to them?
Delegation is custodial (you are transferring funds to a different account, the smart contract that implements staking pool). We provide a reference implementation being security reviewed and tested by 100 validators at time of writing.
We allow validators to write and deploy new contracts but it is up to users to decide if they want to delegate. Validators can compete for delegation by choosing different logic and conditions around tax optimization, etc.
Currently no slashing but will be added as we add shards into the system. At some point validators will be able to add an option to shield delegators from slashing (similar to Tezos model).
### How do we get the balance of an account after it has delegated funds?
One would need to query the staking pool contract to get balance.
## Nodes
### Can a node be configured to archive all blockchain data since genesis?
v
Yes. Start the node using the following command:
```sh
./target/release/near run --archive
```
### Can a node be configured to expose an RPC (ex: HTTP) interface?
Yes. All nodes expose this interface by default which can be configured by setting the value of `listen_addr:port` in the node's `config.json` file.
### Can a node be gracefully terminated and restarted (using archived data on disk to continue syncing)?
Yes.
### Does a node expose an interface for retrieving health telemetry in a structured format (ex: JSON) over RPC?
Yes. `GET /status` and `GET /health` provide this interface.
- `/status`: block height, syncing status, peer count, etc
- `/health`: success/failure if node is up running & progressing
### Can a node be started using a Dockerfile without human supervision?
Yes.
```sh
docker run <port mapping> <mount data folder> <ENV vars> nearprotocol/nearcore:latest
```
See `nearcore/scripts/nodelib.py` for different examples of configuration.
### What is the source of truth for current block height exposed via API?
- MainNet
- https://nearblocks.io
- `https://rpc.mainnet.near.org/status`
- TestNet
- https://testnet.nearblocks.io
- `https://rpc.testnet.near.org/status`
### How old can the referenced block hash be before it's invalid?
There is a genesis parameter which can be discovered for any network using:
```sh
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=EXPERIMENTAL_genesis_config
# in the line above, testnet may be replaced with mainnet or betanet
```
It's `43200` seconds or `~12` hours. You can view the live configuration for `epoch_length` using the [`protocol_config` RPC endpoint](/api/rpc/setup#protocol-config).
In the response we find `transaction_validity_period": 86400` (and since it takes about 1 second to produce a block, this period is about 24 hrs)
## Blockchain
### How will the network will be bootstrapped?
Distribution at genesis will be spread among the NEAR team, our contributors, project partners (ie. contributor, beta applications, infrastructure developers, etc.) and the NEAR foundation (with many portions of that segregated for post-MainNet distribution activity and unavailable to stake so the foundation isn’t able to control the network).
There will be auctions occurring on the platform after launch which will allocate large amounts of tokens over the next 2 years. Additionally we are planning to run TestNet where any validator who participates will receive rewards in real tokens. We are planning to onboard at least 50 separate entities to be validators at launch.
### What is the network upgrade process?
We are currently upgrading via restarting with a new genesis block.
### Which consensus algorithm does NEAR use?
NEAR is a sharded **proof-of-stake** blockchain.
_You can read more in our [Nightshade whitepaper](/docs/Nightshade.pdf)._
> _A few relevant details have been extracted here for convenience:_
>
> [Since NEAR is a sharded blockchain, there are challenges that need to be overcome] including state validity and data
> availability problems. _Nightshade_ is the solution NEAR Protocol is built upon that addresses these issues.
>
> Nightshade uses the heaviest chain consensus. Specifically when a block producer produces a block (see section 3.3), they can collect signatures from other block producers and validators attesting to the previous block. The weight of a block is then the cumulative stake of all the signers whose signatures are included in the block. The weight of a chain is the sum of the block weights.
>
> On top of the heaviest chain consensus we use a finality gadget that uses the attestations to finalize the blocks. To reduce the complexity of the system, we use a finality gadget that doesn’t influence the fork choice rule in any way, and instead only introduces extra slashing conditions, such that once a block is finalized by the finality gadget, a fork is impossible unless a very large percentage of the total stake is slashed.
### How does on-chain transaction finality work?
Finality is deterministic, and requires at least 3 blocks as well as (2/3 +1) signatures of the current validator set.
In a normal operation, we expect this to happen right at 3 blocks but it is not guaranteed.
Finality will be exposed via RPC when querying block or transaction.
Our definition of finality is BOTH:
- Block has quorum pre-commit from the finality gadget. See details of the finality gadget [[here]](/docs/PoST.pdf)
- At least 120 blocks (2-3 minutes) built on top of the block of interest. This is relevant in case of invalid state transition in some shard and provides enough time for state change challenges. In case all shards are tracked and some mechanics to pause across nodes is employed, this is not needed. We recommend exchanges track all shards.
## Accounts
### How are addresses generated?
Please check out the spec here on accounts https://nomicon.io/DataStructures/Account.html.
### What is the balance record-keeping model on the NEAR platform?
NEAR uses an `Account`-based model. All users and contracts are associated with at least 1 account. Each account lives on a single shard. Each account can have multiple keys for signing transactions.
_You can read [more about NEAR accounts here](https://nomicon.io/DataStructures/Account.html)_
### How are user accounts represented on-chain?
Users create accounts with human-readable names (eg `alice`) which can contain multiple keypairs with individual permissions. Accounts can be atomically and securely transferred between parties as a native transaction on the network. Permissions are programmable with smart contracts as well. For example, a lock up contract is just an account with permission on the key that does not allow to transfer funds greater than those unlocked.
### Is there a minimum account balance?
To limit on-chain "dust", accounts (and contracts) are charged a refundable deposit for storing data on the chain. This means that if the balance of the account does not have enough balance to cover an increased deposit for additional storage of data, storing additional data will fail. Also, any user can remove their own account and transfer left over balance to another (beneficiary) account.
There will be a restoration mechanism for accounts removed (or slept) in this way implemented in the future.
### How many keys are used?
An account can have arbitrarily many keys, as long as it has enough tokens for their storage.
### Which balance look-ups exists? What is required?
We have an [RPC method for viewing account](/api/rpc/setup#view_account).
The [JS implementation is here](https://github.com/near/near-api-js/blob/d7f0cb87ec320b723734045a4ee9d17d94574a19/src/providers/json-rpc-provider.ts#L73). Note that in this RPC interface you can specify the finality requirement (whether to query the latest state or finalized state).
For custody purposes, it is recommended not to rely on latest state but only what is finalized.
## Fees
### What is the fee structure for on-chain transactions?
NEAR uses a gas-based model where prices are generally deterministically adjusted based on congestion of the network.
We avoid making changes that are too large through re-sharding by changing number of available shards (and thus throughput).
Accounts don’t have associated resources. Gas amount is predetermined for all transactions except function calls. For function call transactions the user (or more likely the developer) attaches the required amount of gas. If some gas is left over after the function call, it is converted back to NEAR and refunded to the original funding account.
### How do we know how much gas to add to a transaction?
- See reference documentation here: https://nomicon.io/Economics/
- See API documentation for [discovering gas price via RPC here](/api/rpc/setup#gas-price).
The issuer of a transaction should attach some amount of gas by taking a guess at budget which will get the transaction processed. The contract knows how much to fund different cross contract calls. Gas price is calculated and fixed per block, but may change from block to block depending on how full / busy the block is. If blocks become more than half full then gas price increases.
We're also considering adding a max gas price limit.
## Transactions
### How do we follow Tx status?
See related [RPC interface for fetching transaction status here](/api/rpc/setup#transaction-status).
### How are transactions constructed and signed?
Transactions are a collection of related data that is composed and cryptographically signed by the sender using their private key. The related public key is part of the transaction and used for signature verification. Only signed transactions may be sent to the network for processing.
Transactions can be constructed and signed offline. Nodes are not required for signing. We are planning to add optional recent block hash to help prevent various replay attacks.
See [transactions](/concepts/protocol/transactions) in the concepts section of our documentation.
### How is the hash preimage generated? Which fields does the raw transaction consist of?
For a transaction, we sign the hash of the transaction. More specifically, what is signed is the `sha256` of the transaction object serialized in borsh (https://github.com/near/borsh).
### How do transactions work on the NEAR platform?
A `Transaction` is made up of one or more `Action`s. An action can (currently) be one of 8 types: `CreateAccount`,
`DeployContract`, `FunctionCall`, `Transfer`, `Stake`, `AddKey`, `DeleteKey` and `DeleteAccount`. Transactions are composed by a sender and then signed using the private keys of a valid NEAR account to create a `SignedTransaction`. This signed transaction is considered ready to send to the network for processing.
Transactions are received via our JSON-RPC endpoint and routed to the shared where the `sender` account lives. This "home shard" for the sender account is then responsible for processing the transaction and generating related receipts to be applied across the network.
Once received by the network, signed transactions are verified (using the embedded public key of the signer) and transformed into a collection of `Receipt`s, one per action. Receipts are of two types: `Action Receipt` is the most common and represents almost all actions on the network while `Data Receipt` handles the very special case of "a `FunctionCallAction` which includes a Promise". These receipts are then propagated and applied across the network according to the "home shard" rule for all affected receiver accounts.
These receipts are then propagated around the network using the receiver account's "home shard" since each account lives on one and only one shard. Once located on the correct shard, receipts are pulled from a nonce-based [queue](https://nomicon.io/ChainSpec/Transactions#pool-iterator).
Receipts may generate other, new receipts which in turn are propagated around the network until all receipts have been applied. If any action within a transaction fails, the entire transaction is rolled back and any unburnt fees are refunded to the proper accounts.
For more detail, see specs on [`Transactions`](https://nomicon.io/RuntimeSpec/Transactions), [`Actions`](https://nomicon.io/RuntimeSpec/Actions.html), [`Receipts`](https://nomicon.io/RuntimeSpec/Receipts)
### How does NEAR serialize transactions?
We use a simple binary serialization format that's deterministic: https://borsh.io
## Additional Resources
- Whitepaper
- General overview at [The Beginner's Guide to the NEAR Blockchain](https://near.org/blog/the-beginners-guide-to-the-near-blockchain)
- [NEAR Whitepaper](https://pages.near.org/papers/the-official-near-white-paper/)
- Github
- https://www.github.com/near
:::tip Got a question?
<a href="https://stackoverflow.com/questions/tagged/nearprotocol"> Ask it on StackOverflow! </a>
:::
|
---
id: networks
title: NEAR Networks
sidebar_label: Networks
---
NEAR Protocol operates on several networks each operating with their own independent validators and unique state. These networks are as follows:
- [`mainnet`](/concepts/basics/networks#mainnet)
- [`testnet`](/concepts/basics/networks#testnet)
- [`localnet`](/concepts/basics/networks#localnet)
## Mainnet {#mainnet}
`mainnet` is for production ready smart contracts and live token transfers. Contracts ready for `mainnet` should have gone through rigorous testing and independent security reviews if necessary. `mainnet` is the only network where state is guaranteed to persist over time _(subject to the typical security guarantees of the network's validation process)_.
- Status: `https://rpc.mainnet.near.org/status`
- [ [NearBlocks Explorer](https://nearblocks.io) ]
- [ [Wallet](https://wallet.near.org) ]
- [ [Data Snapshots](https://near-nodes.io/intro/node-data-snapshots) ]
## Testnet {#testnet}
`testnet` is a public network and the final testing network for `nearcore` changes before deployment to `mainnet`. `testnet` is intended for testing all aspects of the NEAR platform prior to `mainnet` deployment. From account creation, mock token transfers, development tooling, and smart contract development, the `testnet` environment closely resembles `mainnet` behavior. All `nearcore` changes are deployed as release candidates on first testnet, before the changes are released on `mainnet`. A number of `testnet` validators validate transactions and create new blocks. dApp developers deploy their applications on `testnet` before deploying on `mainnet`. It is important to note that `testnet` has its own transactions and states.
- Status: `https://rpc.testnet.near.org/status`
- [ [Explorer](https://testnet.nearblocks.io) ]
- [ [Wallet](https://testnet.mynearwallet.com/) ]
- [ [Data Snapshots](https://near-nodes.io/intro/node-data-snapshots) ]
## Localnet {#localnet}
`localnet` is intended for developers who want to work with the NEAR platform independent of the public blockchain. You will need to generate nodes yourself. `localnet` gives you the total control over accounts, economics, and other factors for more advanced use cases (including making changes to `nearcore`). For developers, `localnet` is the right choice if you prefer to avoid leaking information about your work during the development process.
More on local development [here](https://near-nodes.io/validator/running-a-node)
`near-cli` [network selection](/tools/near-cli#network-selection) variable is `local`
---
:::tip Got a question?
<a href="https://stackoverflow.com/questions/tagged/nearprotocol">
<h8>Ask it on StackOverflow!</h8>
</a>
:::
|
---
title: 6.3 Metaverse on NEAR
description: NEAR Hub, Octopus App Chains
---
# 6.3 The Metaverse on NEAR: NEAR Hub, Octopus Appchains, and The Role of a Metaverse For An L1
In our final lecture on the Metaverse, we will zoom in specifically on the different Metaverse projects building in the NEAR ecosystem, and also focus on the natural advantages that NEAR may hold to accommodate a Metaverse in the future. We conclude this module on the Metaverse by identifying the role that a Metaverse may play for any L1 in the future, as well as key areas of innovation within Metaverses going forward.
## The Metaverse Projects Currently Building on NEAR
There are two primary Metaverse plays building on NEAR as of January 2023. Interestingly, they each take radically different approaches: The first, is known as NEAR Hub, and is built on NEAR Native, with a specific emphasis on world creation and artificial intelligence integrations. The second, is a Metaverse factory, allowing users to generate their own metaverses as an App-chain on Octopus Network.
### NEAR Hub [Pre-Launch]
Designed and created by Jeff Gold, NEAR Hub is NEAR’s community-developed Metaverse, designed specifically for composability needs within the NEAR Ecosystem.

Similar to Decentraland and Sandbox, Avatar generation and Scene building is included, as are 3D infrastructure of the likes of storefronts or luxury apartments.
What differentiates Near Hub are a number of factors:
* Jitsi based communication enabled inside of the Metaverse (for talking, screen sharing, and calls).
* AI generators capable of creating geNFTs and other forms of AI worked art.
* Built in scene editor, allowing any user to customize a specific scene or parcel of the Metaverse of their choice.
_Business Model:_ The business model for NEAR Hub largely centers around setting fees for use of services, and eventually in providing community, marketing, and socialization services to different dApps inside of the ecosystem. For a dApp on NEAR, the value proposition of having a parcel of the Metaverse, or a place inside of it is exposure specifically to the NEAR community that accesses that Metaverse. The question remains (addressed fully below), to what extent is an active community inside of an L1 ecosystem, a necessary prerequisite for a successful Metaverse to thrive?
### Reality Chain
Significantly different from NEAR Hub, is Reality Chain. Reality Chain takes an entirely different approach to the Metaverse. Launched as an Appchain on Octopus Network (discussed in Module 9) Reality Chain brands itself as a Metaverse generator, such that any builder can create their own custom Metaverse using the tooling and specifications of Reality chain.

Branded as a _social metaverse_, Reality Chain limits itself to attempting to create a more enhanced and immersive social experience, that does not attempt to over-intrude:
_“Reality Chain is an award-winning Social Metaverse that seeks to give you a non-immersive Metaverse experience with the power of Web 3.0. A Metaverse shouldn’t replace your social interactions; and instead, it should be a complementary part of your social life. Reality Chain’s Metaverse provides a “casual” experience while still giving you the benefit of owning pieces of it as NFTs.” ([Reality Chain](https://www.realitychain.io/))_
Monetarily, the $REAL token is utilized to mint land and parcels for anyone looking to create their own social Metaverse. To date, the most successful dApp built on top of Reality Chain is known as [Myriad Social - a social network that is attempting to create a Metaverse component to its service. ](https://www.myriad.social/myriad-metaverse)
## Natural Advantages for NEAR: Social, Account Model, and More
Looking ahead into the future, there are a number of opportunities for NEAR to capitalize on the future emergence of the Metaverse, specifically centering on unique design propositions of the NEAR Ecosystem:
* **Cheap Transaction Costs:** Unlike Ethereum, which averages a transaction cost from between $8 to $50 dollars (depending on network congestion), NEAR can sustainably handle millions of users at a time, at a cheap and cost effective rate. This is evident but should be emphasized specifically for any thriving metaverse.
* **[NEAR.Social:](https://near.social/#/)** NEAR already has its own social-network, capable of establishing profiles, identity, and communication between users. This as an open-source starting point is already providing the groundwork for pulling NEAR social into any Metaverse of the future.
* **The NEAR Account Model:** The inbuilt NEAR account model, provides the equivalent of a domain service for the NEAR ecosystem. While currently naming is only possible with a name.near ID, subaccounts, as well as new high level accounts name.usa, name.australia, etc. are in principle also possible. This is an optimal in-baked logic, for the future identification of metaverse avatars, the buying and selling of accounts that own metaverse real estate, and the seamless onboarding of new users into the Metaverse with a familiar name ID.
* **Robust DAO Tooling and Role Based Decision Making via Astro DAO:** Metaverse governance, collective decision making, and partitioning of ownership over certain facets of the Metaverse, via Astro DAO and Sputnik DAO, create an easy plug-and-play type model from which decentralized governance can be directly imbued into any or all of a given metaverse.
## The Role of a Metaverse For An L1
As crypto continues to grow and mature, the place of the Metaverse in a given ecosystem remains an untapped super-dApp that has yet to be successfully tapped into.
This final, closing part of our treatment of the Metaverse zooms into the _macro trends_ surrounding its development into the future, and specifically its intended function in an L1 ecosystem.
To start, let's imagine a robust L1 ecosystem, crawling with users across a diverse product stack of DeFi protocols, storage solutions, NFT Marketplaces, practical NFTs (music, fashion, etc.), a number of diverse communities, ecosystem wide governance, and a sizable portion of ecosystem generated value. In short, an L1 ecosystem that is thriving with users, value, and activity.
### What does a Metaverse do in this situation?
Simply put, it provides an aggregated interface and platform for easing transaction costs, improving exposure among dApps, strengthening community - including visibility of OG community members or reputable leaders, and creating an all-in-one interface for visibility into the larger ecosystem at hand.
By establishing property and ownership inside of this Metaverse, it establishes a further level of permanence that can later on be connected with the physical world via hardware (glasses, headsets, physical locations and portals into areas of the Metaverse, and so forth).
Remembering that we are early to the concept of virtual worlds and open-decentralized community operated Metaverses, this has nevertheless led industry predictors to see growth in the Metaverse as an [industry cluster in the hundreds of billions of dollars](https://www.globaldata.com/store/report/metaverse-market-analysis/#:~:text=According%20to%20GlobalData%20estimates%2C%20the,crucial%20technologies%20driving%20its%20development.) over the coming 7 years.
A full thesis is outlined on this topic [by Delphi Digital](https://naavik.co/deep-dives/into-the-void), but the most important high level takeaway - and in line with what was discussed in lecture 1 of this module can be summarized as the following:
_“The Metaverse Represents Our Next Great Milestone, As A Networked Species.” ([Naavik](https://naavik.co/deep-dives/into-the-void))_
## Key Areas of Innovation in the Metaverse
In the NEAR future, we can imagine innovation in further developing the metaverse in the following manners:
* _Metaverse Complexity:_ Whereby scarcity of parcels, access to certain areas of a metaverse, and the capacity to create items inside of the metaverse, is gamified further through metaverse native elements or building blocks that can be extracted and harvested at scale. Conversely, this also applies to requiring certain thresholds or access levels, to different areas in the metaverse, either based on a reputation system or through staking certain amounts of a token.
* _Composable Integrations and dApps:_ Integrating different dApps, generators, and marketplaces from an L1 ecosystem, into the Metaverse, such that it becomes an ‘integrated’ and ‘composable’ product, as opposed to a stand alone isolated product.
* _Exclusive Launches and Events:_ Hosting unique and rare events in the Metaverse, including original launches of PFP collections, music album releases, special events and announcements, and even ecosystem wide elections.
* _AR/VR Integration:_ Creating a symbiosis between the digital and the physical world, such that physical hardware can enhance the digital experience, and digital locations can be specific to physical areas (Pokemon Go style).
* _AI / Crypto Synergies:_ [Bridging the gap](https://www.intechopen.com/chapters/77823) between crypto and AI, the Metaverse offers an ideal and holistic platform for AI generators to be inserted for use.
* _Crypto-Economic Design:_ Increasing the sophistication of Metaverse crypto-economic systems, such that synergies can be established between the mother ecosystem hosting the metaverse (the L1 token in question), and conversely, partner dApps with their own tokens, that can be used within the Metaverse. In the long-term future, this would also relate to bringing in new types of value to gamified features of the Metaverse (carbon credits for example), and overlaying different tokens (both fungible and non-fungible) into the underlying world and mechanism design for users to participate in. |
NEAR & Gaming: A Deep Dive into OP Games
COMMUNITY
August 15, 2022
Alongside DeFi and NFTs, Web3 gaming is proving to be one of the most vibrant and creative spaces in crypto. From play-and-earn titles to fractionalized game ownership, crypto gaming’s moment is now. And it’s evolving in real-time.
OP Games, arguably the most successful gaming company building on NEAR right now. With Arcadia, its aptly-named community-owned arcade, a plug-and-play developer SDK, and monetization features, OP Games is revolutionizing gaming with Web3 technologies.
In the latest installment of our 4-part series “NEAR & Gaming”, we take a deep dive into OP Games. Not only does OP Games have some lessons for developers building during a down market, they have offer up some useful ideas for onboarding Web2 users into the Web3 world, and more.
Born in the crypto bear market of 2018
While OPGames is a major gaming platform on NEAR, its origins weren’t exactly auspicious. Growing out of the mobile gaming company Alto, OPGames emerged in the crucible of 2018’s Great Crypto Bear Market.
Co-founders Chase Freo (CEO) and Paul Gadi (CTO) had a background in Web2 gaming and on other tech projects. A game publisher and investor, Freo grew disillusioned with the 70/30 revenue split that was standard for game publishers and designers. But it was through their work in Web2 gaming that Freo and Gadi, and one of the other original Alto founders, Gabby Dizon, came to know each other.
“We realized we aligned in terms of how gaming would work outside of the traditional [mobile and desktop]space,” says Freo. “We also realized we were all into blockchain and crypto, so we co-founded OP Games, which was called Alto at the time.”
Given the bear market of the period, Freo and Gadi started building a lot of different products, especially in the NFT space. At the time, Web3 developer funds were hard to come by. Freo and Gadi watched as Dapper Labs and The Sandbox got funding, and thought these were good signs.
While funding didn’t immediately materialize for OP Games, the pair’s work on NFTs was not in vain. Indeed, that work convinced Freo and Gadi of OP Games’ direction and, ultimately, led them to NEAR.
“There’s a certain way game developers make money in Web2 and it’s skewed towards bigger game companies, bigger game developers, and bigger game publishers,” Freo explains. Developers gravitate toward publishers, who can distribute the game and onboard millions of players. Unless, of course, a game goes viral, like Flappy Bird—which is rare.
“Because of this extreme reliance on publishers, the deal flows became very one-sided,” says Freo. “And that made me question if I was doing anybody a favor by being a game publisher. That changes the power structure in terms of how much a game developer earns versus how much a publisher earns, and how then how much Apple and Google earn. So there’s a lot of gatekeeping involved.”
What Freo and Gadi saw in Web3 was elegantly simple and profound: the democratization of gaming. Each player had their own wallet and developers didn’t need one million players to succeed.
“Most players in Web3 are also paying players via NFTs, right,” Freo explains. “Obviously, there’s a lot of experimentation involved in play-to-earn, which has now evolved to play-and-earn. But we’re hopeful and we’re always thinking about newer models that will benefit people in the long run.”
Building OPGames for other games developers
During the 2018/2019 crypto downturn, OP Games received grants from a few different entities. Freo says there is a tendency to spend funds on an entire product suite. “Build the dream”, as he refers to it. But Freo and Gadi took another approach.
“We realized very quickly that we have to be very modular in terms of what we’re building, so that we can still continuously create something, even though it’s at a much slower pace,” says Freo. “But it would allow us to create multiple things that would be useful right away in that period.”
Freo compares the OP Games platform’s modularity to Legos: it gives developers all of the pieces they need to design and launch successful Web3 games. Early on in this slow build process, Freo and Gadi developed SDKs and APIs that game developers could use in their own slow builds.
“We modularize how we want to build,” says Freo. “We want to make sure that each and every single one of these product lines will be as useful now as it will be in the future. And once we succeeded in building these products, it was much easier to fundraise when the bull market was in full swing.”
“We’re building the supply of games in Web3 by bringing Web2 game developers into the mix,” he adds. “And we have this tooling, like Legos, that they can use right away. It’s simplified so that it’s easy for their games to integrate or reap the benefits of a Web3 token offer.”
A look inside the OP Games feature set
After pivoting into Web3 gaming, OP Games began developing its first feature—the “Tournaments” function. This feature allowed any Javascript game to use OP Games’s JS SDK, creating a plug-and-play environment asynchronous play.
“Game developers could now set up their own tournaments on a daily or monthly basis, or however they wished to do it,” Freo explains. “They could set up parameters in terms of what the pot would look like, what percentage would go back to players and developers, and so on and so forth.”
OP Games next created Arcadia.fun, a Web3 game arcade where game designers could load their games using the SDK. Currently in Alpha, Arcadia will soon get an official launch with a dedicated website. To date, there are 43 games on Arcadia, including one of OP Games own titles, Arcadeum Arena, a hybrid auto-chess player, avatar-battler.
The team also built a Leaderboard feature that showcased the top players for any given game. After launching Tournaments, Arcadia, and Leaderboard, the team turned their attention to a player-versus-player (PvP) feature. So, instead of playing against a game’s artificial intelligence, OP Games players could play against each other.
“Currently we’re working on NFT wagering,” says Freo. “This will allow players to wager their NFTs against each other. And we fulfill that position based on whomever wins a specific game on the Arcadia platform. On the back end there are these little feature sets that we’ve built for developers to use that they can plug into their game so that they can exist in Arcadia.”
To make this all happen, OP Games allows players to log in with their NEAR wallet. This makes it possible for the gaming platform to offer $NEAR as a buy-in and pay-out for winnings. While NEAR’s technical advantages made it the obvious choice for OP Games development, Freo and Gadi envision OP Games as ultimately blockchain agnostic. A multi-chain, Open Web gaming platform that can help onboard the masses into the larger Web3 ecosystem.
“All of these games can be played on the web or on a mobile-optimized web,” says Freo. “That’s where we’re at at the moment. And we’re still building a few things, specifically in our marketplace, and a much bigger metagame for the platform itself,. We will be launching that game before the end of the year.”
Welcoming Web2 developers into crypto gaming
Rust and Solidity are great programming languages for Web3 development. But Freo notes that the vast majority of developers design games using Unity (C++), Javascript, Swift, and others.
Which is why NEAR’s forthcoming Javascript SDK will be a game changer in making the NEAR ecosystem platforms like OP Games great creative centers for Web2 and Web3 developers alike.
“A big chunk of these game developers that we’re working with are actually not crypto-native,” says Freo. “Many designers wanted to move into crypto and take advantage of the promise of Web3, but they don’t know exactly where to start.”
“Traditionally, they’re building on open source Web3 game engines that support Javascript and on Unity as well,” he adds. “I think about 80% of games are being built in Unity. “
NEAR’s Javascript SDK will help attract Web3 game developers. But, going forward, that will be only part of the work toward capitalizing on Web3’s democratizing power. To make development even easier, Freo says it’s vital to optimize game development on NEAR using Rust.
“Within Human Guild, which I’m also part of, we’re trying to invest in a lot of infrastructure to make sure that we’re able to facilitate game development in Rust,” he says. “And on the infrastructure level, NEAR is working to make sure that there is support for all game developers.”
|
---
sidebar_position: 4
sidebar_label: "Linkdrop contract"
title: "Introducing the linkdrop contract we can use"
---
import {Github} from "@site/src/components/codetabs"
import createMainnetAccount from '/docs/assets/crosswords/create-mainnet-account.png';
import createTestnetAccount from '/docs/assets/crosswords/create-testnet-wallet-account.png';
# The linkdrop contract
We're going to take a small detour to talk about the linkdrop smart contract. It's best that we first understand this contract and its purpose, then discuss calling a method on this contract.
[The linkdrop contract](https://github.com/near/near-linkdrop) is deployed to the accounts `testnet` and `near`, which are known as the top-level accounts of the testnet and mainnet network, respectively. (Anyone can create a linkdrop-style contract elsewhere, but the one shown here is the main one that others are patterned off of.)
## Testnet
There’s nothing special about testnet accounts; there is no real-world cost to you as a developer when creating testnet accounts, so feel free to create or delete at your convenience.
When a user signs up for a testnet account on NEAR Wallet, they'll see this:
<img src={createTestnetAccount} width="400" />
Let's discuss how this testnet account gets created.
Notice the new account will end in `.testnet`. This is because the account `testnet` will create a subaccount (like we learned about [earlier in this tutorial](../01-basics/02-add-functions-call.md#create-a-subaccount)) called `vacant-name.testnet`.
There are two ways to create this subaccount:
1. Use a full-access key for the account `testnet` to sign a transaction with the `CreateAccount` Action.
2. In a smart contract deployed to the `testnet` account, call the `CreateAccount` Action, which is an async method that returns a Promise. (More info about writing a [`CreateAccount` Promise](/sdk/rust/promises/create-account).)
(In the example below that uses NEAR CLI to create a new account, it's calling `CreateAccount` on the linkdrop contract that is deployed to the top level "near" account on mainnet.)
## Mainnet
On mainnet, the account `near` also has the linkdrop contract deployed to it.
Using NEAR CLI, a person can create a mainnet account by calling the linkdrop contract, like shown below:
<img src={createMainnetAccount} />
The above command calls the `create_account` method on the account `near`, and would create `aloha.near` **if it's available**, funding it with 15 Ⓝ.
We'll want to write a smart contract that calls that same method. However, things get interesting because it's possible `aloha.near` is already taken, so we'll need to learn how to handle that.
## A simple callback
### The `create_account` method
Here, we'll show the implementation of the `create_account` method. Note the `#[payable]` macro, which allows this function to accept an attached deposit. (Remember in the CLI command we were attaching 15 Ⓝ.)
<Github language="rust" start="125" end="149" url="https://github.com/near/near-linkdrop/blob/ba94a9c7292d3b48a0a8ba380fb0e7ff6b24efc6/src/lib.rs" />
The most important part of the snippet above is around the middle where there's:
```rs
Promise::new(...)
...
.then(
Self::ext(env::current_account_id())
.on_account_created(...)
)
```
This translates to, "we're going to attempt to perform an Action, and when we're done, please call myself at the method `on_account_created` so we can see how that went."
:::caution This doesn't work
Not infrequently, developers will attempt to do this in a smart contract:
```rust
let creation_result = Promise::new("aloha.mike.near")
.create_account();
// Check creation_result variable (can't do it!)
if creation_result {...}
```
In other programming languages promises might work like this, but we must use callbacks instead.
:::
### The callback
Now let's look at the callback:
<Github language="rust" start="151" end="164" url="https://github.com/near/near-linkdrop/blob/ba94a9c7292d3b48a0a8ba380fb0e7ff6b24efc6/src/lib.rs" />
This calls the private helper method `is_promise_success`, which basically checks to see that there was only one promise result, because we only attempted one Promise:
<Github language="rust" start="32" end="42" url="https://github.com/near/near-linkdrop/blob/ba94a9c7292d3b48a0a8ba380fb0e7ff6b24efc6/src/lib.rs" />
Note that the callback returns a boolean. This means when we modify our crossword puzzle to call the linkdrop contract on `testnet`, we'll be able to determine if the account creation succeeded or failed.
And that's it! Now we've seen a method and a callback in action for a simple contract.
:::tip This is important
Understanding cross-contract calls and callbacks is quite important in smart contract development.
Since NEAR's transactions are asynchronous, the use of callbacks may be a new paradigm shift for smart contract developers from other ecosystems.
Feel free to dig into the linkdrop contract and play with the ideas presented in this section.
There are two additional examples that are helpful to look at:
1. [High-level cross-contract calls](https://github.com/near/near-sdk-rs/blob/master/examples/cross-contract-calls/high-level/src/lib.rs) — this is similar what we've seen in the linkdrop contract.
2. [Low-level cross-contract calls](https://github.com/near/near-sdk-rs/blob/master/examples/cross-contract-calls/low-level/src/lib.rs) — a different approach where you don't use the traits we mentioned.
:::
---
Next we'll modify the crossword puzzle contract to check for the signer's public key, which is how we now determine if they solved the puzzle correctly.
|
---
id: how-to-contribute
title: How to Contribute
sidebar_label: How to Contribute
sidebar_position: 3
---
## Ways to Contribute to the code {#ways-to-contribute-to-the-code}
If you happen to notice any bugs, open an issue. If you have a more involved suggestion for the NEAR Protocol standard, we encourage you to submit a [NEAR enhancement proposal](https://github.com/nearprotocol/NEPs) \(NEPs\).
Take a look at existing issues in our various repo's to see what you can work on. If you're interested in working on an issue, we recommend you comment on it so that a maintainer can bring you up to speed, and so that others know you are working on it.
If there's something you'd like to work on, but isn't captured by an existing issue, feel free to open an issue to start that discussion!
We'll always need help improving documentation, creating tutorials, and writing tests.
## Open Issues in NEAR repositories {#open-issues-in-near-repositories}
There are [various repo's](https://github.com/near) you can contribute to. If you're looking to collaborate and want to find easy tasks to start, look at the issues we marked as ["Good first issue"](https://github.com/search?q=org%3Anearprotocol+is%3Aopen+is%3Aissue+label%3A%22good+first+issue%22&unscoped_q=is%3Aopen+is%3Aissue+label%3A%22good+first+issue%22).
## Creating and submitting a Pull Request {#creating-and-submitting-a-pull-request}
As a contributor, you are expected to fork a repository, work on your own fork and then submit a pull request. The pull request will be reviewed and eventually merged into the main repo. See ["Fork-a-Repo"](https://help.github.com/articles/fork-a-repo/) for how this works.
## A typical workflow {#a-typical-workflow}
Make sure your fork is up to date with the main repository:
```text
cd near-core
git remote add upstream https://github.com/near/nearcore.git
git fetch upstream
git pull --rebase upstream master
```
**NOTE: The directory near-core represents your fork's local copy.**
Branch out from `master` into `fix/some-bug-#123`: \(Postfixing \#123 will associate your PR with the issue \#123 and make everyone's life easier =D\)
```text
git checkout -b fix/some-bug-#123
```
Make your changes, add your files, commit and push to your fork.
```text
git add SomeFile.js
git commit "Fix some bug #123"
git push origin fix/some-bug-#123
```
Go to [https://github.com/near/nearcore](https://github.com/near/nearcore) in your web browser and issue a new pull request.
Read the PR template very carefully and make sure to follow all the instructions. These instructions refer to some very important conditions that your PR must meet in order to be accepted, such as making sure that all tests pass, etc.
Maintainers will review your code and possibly ask for changes before your code is pulled in to the main repository. We'll check that all tests pass, review the coding style, and check for general code correctness. If everything is OK, we'll merge your pull request and your code will be part of the NEAR repo.
Please pay attention to the maintainer's feedback, since its a necessary step to keep up with the standards NEAR attains to.
## All set! {#all-set}
If you have any questions feel free to post them to our [discord channel](http://near.chat).
Thanks for your time and code!
:::note Got a question?
**[Ask it on StackOverflow!](https://stackoverflow.com/questions/tagged/nearprotocol)**
:::
|
NFTs on NEAR: Beyond the Hype
COMMUNITY
May 16, 2022
Soaring both in growth and interest in 2022, the Open Web movement has become a cultural zeitgeist. Cryptocurrency and blockchain, critical to reimagining the web, are together championed as the remedy to our currently broken global economy. Without argument, 2021 was the year of the non-fungible token, more commonly known by its now ubiquitous acronym, NFT. Sales of NFTs surged to $17.7B in the last year, up from $82.5M in 2020—a 200-fold increase.
NFTs have permeated all corners of today’s society and are here to stay. According to Google Trends, “NFT” surpassed “crypto” in search queries in December 2021. Bored Ape Yacht Club NFTs continue to sell to a slew of celebrities for millions; footwear and apparel behemoth NIKE acquired a dedicated NFT team in 2021; Saturday Night Live even featured a skit on the subject.
While it’s clear that NFTs are mainstream culture, beneath the hype lies a powerful piece of malleable technology. NFTs are so much more than exclusive pictures recorded on the blockchain. They are also more than collectibles hung on the digital gallery walls of their owner’s wallet.
Developers are leveraging NFTs on NEAR to create artist storefronts, power gaming platforms, release music, and fund vertical farms in urban spaces. And that’s just the beginning.
Minting NFTs cheaper, faster, and securely
Every NFT starts as an idea. The journey that idea must take to become an NFT used to be a convoluted one. It required technical prowess from the end user and was costly to mint. This journey was elegantly simplified by Mintbase, a global platform that allows anyone to create NFTs without developer skills.
As the “Shopify for NFTs,” people use Mintbase’s platform to create and sell music, art, memberships, photography, and so much more. Event organizers can even create NFTs to sell tickets for their next event.
Creating a digital storefront is the first step to minting NFTs on Mintbase. To do so, a user creates a smart contract and pays a gas fee. This is typically the most costly part of the process, especially on Ethereum, where gas fees are high from network congestion.
NEAR gas fees are impressively lower than Ethereum’s. NEAR features a more efficient contract execution model that requires less computation and uses the Nightshade dynamic sharding approach, making the network infinitely scalable. On Mintbase, deploying a store and minting NFTs is now significantly cheaper and more accessible to a diverse population of creators.
Building the future of gaming, one block at a time
OP Games, a Web3 gaming arcade platform, combines the worlds of gaming and NFTs. The fusino is creating a radical transformation in gameplay that gives both power and control back to the players. Using fractionalized NFTs, OP Games’ HTML5 arcade allows the community to effectively own a piece of the games they play.
With this type of collective ownership, the OP Games community will see NFT price appreciation as any game grows and becomes more successful. This effectively monetizes their time and attention during gameplay. Furthermore, the OP Games DAO (decentralized autonomous organization) establishes a relationship between the players and developers, giving the former a voice in how the game evolves over time.
Through the OP Games DAO, the community can ask a game’s developers to create different levels and new in-game assets that they can buy, own, and trade while playing. Whereas most gaming platforms lock digital collectibles in their own ecosystem, OP Games users can bring in accomplishments and skins from other games. This gives players more meaningful rewards for the time they invest in virtual worlds—all made possible through NFTs.
Reinventing the record label with NFTs
DAO Records uses NFTs to drastically reimagine what a record label can be. Building tools to help write the future of the music industry, the label is pushing the not-so-radical idea that musicians should be fairly compensated for their songs. DAO Records disrupts the antiquated recording industry on multiple fronts. Its founders see the organization as an audio NFT distribution platform and virtual event service for artists of all kinds.
Founders Vandal and DJ Lethal Skillz are encouraging artists to release and package new music as audio NFTs, whose smart contracts allow for an entirely more equitable royalty structure in perpetuity. Indie artists of any genre can easily mint audio NFTs and release their music. DAO Records sees the future of the music industry as a place when musicians control their work as well as their relationship with fans.
The label released its first audio NFT in February 2020: a gold edition of a cover art collectible and unlockable WAV file. Two months later, DAORecords pushed audio NFT boundaries even further with the release of their first official interactive audio NFT. Titled Mint Tape Vol 1, the NFT is a USB cassette of 25 songs and 30 accompanying artworks. Since its inception, DAO Records has released 350+ NFTs from over 100 artists.
Beyond NFT releases, DAO Records has held over 50 virtual events in their cryptovoxel space, “The Playground.” Their goal is to build a lively, dynamic metaverse community for music and art-related virtual events in The Playground and other custom-built virtual venues.
Changing the way we connect with food
Lisbon-based Raiz uses NFTs to help fund vertical farms—the practice of growing crops in vertically stacked layers—in under-utilized urban spaces. Its mission is to transform urban areas into epicenters for the growing and harvesting of foods for local communities and restaurants. Founded by Emiliano Guitterez, Raiz is ardently opposed to the agriculture industry’s stagnant business model, which ships harvested food across global markets.
Raiz is bringing renewed focus back to the local community while maintaining incredible taste and nutrients in their crops. With this approach, the team thinks it can disrupt the current unsustainable model. Raiz’s vertical farming model also incorporates controlled-environment agriculture, optimizing plant growth through sustainable technology that directly impacts our planet.
Raiz uses NEAR-powered NFTs to generate yield and offer “tokenized impact for the bold.” Through NFTs, Raiz makes and releases digital artworks of plants linked to impact metrics such as carbon emissions and water savings—significant issues in traditional agriculture and industrial farming markets.
Raiz is transforming local hydroponic, vertical farming, and solar energy systems into investable crypto assets by linking the physical with the digital. Raiz’s NFT model can be used for other local food-growing efforts as well, giving people greater access to tokenized environmental and local impact.
NFTs as a gateway, not a barrier
People, ideas, and projects built on NEAR operate on the new frontier of Web3 technology. Now creators, developers, business owners, and individuals can leave their legacy in the digital world through NFTs. Low gas fees and fast transactions combined with a no-compromises approach to security make NEAR Protocol the ideal platform. NEAR empowers these creators, who can directly engage with their fans and end users, accomplishing new feats through NFTs.
NFTs are producing a giant leap in transparency and accountability when distributing content, running a business, or supporting a cause. All of the backend accounting is done transparently on the blockchain. It is visible on NEAR Explorer, allowing for transparency paired with an actual ability to illustrate the flow of funds.
Royalties on NEAR follow the NFTs in perpetuity, allowing for royalty enforcement across different marketplaces and into secondary sales. With the royalties embedded in the NFT token, they must be respected to complete the transfer of the NFT. With complete customization, royalties can be split between multiple contributors, beneficiaries, and DAOs. This standard is the first of its kind and a key differentiator for NFT developers, artists, and users alike.
Thanks to NEAR’s robust framework and infrastructure, the greatest barrier is the capacity to innovate instead of the technology itself. Supporting documentation and open source repositories for building NFT marketplaces on NEAR are available to all. Any developer can build on these concepts and choose different elements to get brand new ideas off the ground. Any user can translate an idea to an NFT seamlessly. A low barrier to entry for developers and creators translates to massive exposure and accessibility to users.
Beyond the hype, NFTs are transforming multiple industries across today’s landscape. Creators are gaining momentum and disrupting the status quo through seamless and easy tech, all powered on NEAR. |
On usability of blockchain applications
DEVELOPERS
April 17, 2019
On usability of blockchain applications
A scalable blockchain in the modern world is like a stadium in the middle of a desert: it has a lot of seats, but nobody to sit on them
Imagine you want to play a blockchain game. For example, say you want to get a cryptokitty. Or play some collectible card game. It is actually a pretty involved process. You need to:
Install Metamask;
Create a key pair, and securely store the private key; If you want to later play from another device, you need to understand how to transfer it to that device;
Register on Coinbase;
Do a KYC, which involves sending your documents to Coinbase;
Wait for a few days;
Buy Ether. Yes, you need to make a purchase before you can even try the game!
Transfer Ether from Coinbase;
Finally, buy your kitty! Though now you need to pay for every interaction with the game, and your latency is at least 20 seconds.
Modern games and applications running on blockchains report a whopping 95–97% drop-off rate during the above onboarding process. That means that out of 100 users who try the application only 5 or fewer actually get to start using it!
The problem above can be roughly split into three subproblems:
The necessity to install a browser plugin (or have a wallet application) to securely interact with the chain;
The necessity to have and understand public/private keys security;
The necessity to pay for gas for each transaction.
Items 1 and 2 above are mandatory for interacting with the blockchain securely, they are designed to make sure the user doesn’t lose their funds or assets. The last item, besides providing a financial incentive to the miners, is also necessary to keep the blockchain itself secure — if transactions were free, it would be trivial to DDoS the system by spamming it with lots of free useless transactions.
Once a person is involved in a particular blockchain ecosystem, such as Ethereum or NEAR, they do have the browser plugin or wallet installed, have some assets on their accounts, and have all their devices set up to use the proper key pairs. For them using Web3 applications is relatively easy, besides maybe the fact that the applications are slow (latency and throughput of blockchain applications are beyond the scope of this writeup, check out our previous posts on sharding: one and two, as well as tech deep dives with developers of plasma: one and two, and state channels).
However, as of today, the majority of internet users do not use blockchain, and if we want it to change, we need to make the onboarding for them as streamlined as possible. In the ideal world developing a decentralized application running on a blockchain shall be no harder than building a nodeJS application, and once such an application is deployed, a user that never used blockchain before should be able to just open it in a browser and start interacting with it.
Let’s consider each of the barriers described above, what efforts are made to fix them today, and what changes we are developing on the protocol level to support them.
If you prefer video, you can watch me giving a talk at Berkeley on the same topic here:
Browser plugins / Wallet apps
You do need some custom binary running on your machine to securely interact with the blockchain. The motivation behind it is that anything hosted that you just open in your browser is completely controlled by the host, and thus can be arbitrarily changed at any point. Even if the hosted solution stores the keys locally encrypted, the code of it can later be changed to fetch the data from the local storage and send it to the remote server immediately after it was decrypted to be used for some interaction with the blockchain.
However, consider a person not involved in blockchain today buying crypto. Are they likely to set up their account locally and store funds there, or just store them on Coinbase, which is a completely centralized service? They will probably choose the latter.
Similar reasoning shall apply to use decentralized applications. When the user starts interacting with the blockchain they shall be able to do that through a hosted solution. It will provide lower security, since the centralized entity will have an ability to take over the account, but early on the user doesn’t have much to lose, so much security is no worse than what one gets today with centralized services to whom the users trust a great deal of their assets and data.
To emphasize this point, observe that most people install MetaMask from the Firefox or Chrome extensions catalog, and wallet applications from iTunes or PlayStore, effectively trusting both the MetaMask / wallet applications developers, and some big player such Mozilla, Apple or Google. It is extremely rare for one to install MetaMask from source, after carefully examining the code. Thus we already trust the security of our accounts to centralized entities.
There are solutions developed today that developers can integrate into their decentralized applications that would make it possible to interact with the application without installing browser plugins and wallet applications, such as Portis. The problem with such services is that once one trusted their private key to such a service, ultimately the security of the account is permanently compromised. If one later wants to get the full ownership over the account, they must create a new account and transfer all assets to such an account. If a particular application doesn’t provide a convenient way to transfer assets, the user will never be able to gain full ownership over such assets.
One solution to this problem is to have a contract-based account such that the user can replace the key that controls the account once they wish to do so. But for this to work the account needs to be contract-based from day one, and unless Portis or other service creates such a contract based account by default, users will not have this ability. Further, contract-based accounts cannot do everything that a regular account protected by a private key can do.
In NEAR each account is contract based by default, and a hosted wallet is provided by NEAR. Ultimately the user can start interacting with the blockchain by using the hosted wallet, and then later at any instance update the security of the account by creating a new key pair locally and updating the account to use such a key pair.
Someone suggested a term we like a lot for this approach: progressive security. The user transitions from the highest usability and low security to the highest security and low usability over time, as their involvement and investment into the blockchain increases.
Understanding private/public key pairs
If we convinced you that progressive security is a good thing, and hosted wallets are a way to go, key pairs are gone naturally. In the simplest approach, the hosted wallet stores the private keys of all the users in its own hosted database, and provide its own authentication layer to the users. It can offer them to use Facebook login, Google login, or just good old email and password option. Only once the user wants to transition from using the hosted wallet do they need to set up a private key properly, and learn how to transfer it to other devices.
Interestingly, with the contract based accounts, the transfer process itself can be done easier while maintaining the full security. Instead of transferring the private key to another device via some insecure channel, the contract that governs user’s account can have two methods: `proposeNewSk` and `approveSk`, where the first method can be invoked by anyone, and adds a new private key into a list of proposed private keys for the account, and `approveSk` can only be called with a signature from one of the existing private keys on the account, and can approve any of the proposed private keys for the account. This way a user can set up a brand new key pair on the new device, propose the new private key from such device, and approve it from the existing device.
Gas fees
Any transaction that is executed on chain consumes a somewhat large amount of resources. For a state change to be executed securely, a large number of independent entities need to validate the state transition before it is applied. Since there’s some amount of resources spent on executing the transaction, it cannot be free.
When one compares web3 to web2, they often argue that web2 services by nature are free today. One doesn’t pay for every transaction when they use Facebook. But in reality, they do. Facebook would not provide a free service to the users if the expected long term value from the user didn’t exceed the resources spent on the resources spent processing their requests and storing their data, as well as the cost of acquiring such a user. When using Facebook, users both pay with their data, access to which Facebook then abuses in the most unacceptable ways, and with their attention. The following screenshot literally doesn’t have a single block of information that is not sponsored:
(the value of x is 2)
In the case of the blockchain, if an application developer believes that the total lifetime value from the user will exceed the gas cost for their transaction, they shall be able to pay for such transactions. It is one of the few ideas that come from EOS that makes a lot of sense. Similarly, if the hosted wallet has some value in users using the applications, they can choose to cover the costs as well. For example, NEAR might opt in to cover some gas costs for each user, since it is highly motivated to get higher adoption for the protocol. CryptoKitties can choose to cover the cost for interactions with their contracts, since users that start playing CryptoKitties are very likely to buy one, and the expected value of a user is extremely high.
This only solves one part of the puzzle: offsetting the costs of executing transactions. If users don’t have to pay for transactions, they can spam the system with free transactions, and saturate the allowance that the hosted wallet or the application developers set for free usage. But similarly, people who use Facebook can spam them with free requests and saturate their resources. This problem is by no means unique to blockchain and has plenty of solutions already existing. The hosted wallet can choose to implement one such DDoS prevention solution, and still provide users with free transactions.
There’s still a problem. The model in which someone pays for the user expecting some value from them later is easily abusable. There’s a reason why Google, Facebook, Apple, and other tech giants have non-transparent privacy policies and completely disrespect users’ privacy. The entire motivation behind web3 is to put an end to such practices, but the very way we try to attract users promotes such practices again.
There’s however a fundamental difference. In web3, while the user can start using a service paying with the future expected value, they can at any point switch to paying for transactions themselves and use a hosted wallet, or a browser extension, that doesn’t try to take any advantage of the user’s privacy.
Outro
With the progressive security concept and particular solutions above, we can provide users with the onboarding as simple as it is today in web2, with an ability to upgrade to the full blockchain security at any moment in the future.
We are writing a separate blog post on the other side of the problem: ease of development. The state of developers experience in Ethereum is far from perfect, and we believe that it can be improved significantly.
While waiting for the blog post, you can already experiment with our development experience. Try out our online IDE, and read the documentation.
NEAR Protocol builds a sharded proof of stake blockchain with a fanatical emphasis on usability. If we intrigued you, please follow us on Twitter, and join our Discord, where we discuss all the topics related to tech, economy, governance and more.
https://upscri.be/633436/ |
---
id: what-is
title: What is Chain Abstraction?
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
import {CodeTabs, Language, Github} from "@site/src/components/codetabs"
The idea behind `chain abstraction` is quite simple: **blockchain** technology should be **abstracted away** from the user experience. In other words, people should **not realize** when they are **using a blockchain**, nor **which blockchain** they are using.

To help on this task, NEAR Protocol provides services that allow to **create** and **recover accounts** using **email addresses**, use the account **without acquiring funds**, and **control accounts** in **other chains**. All in the most **seamless** way possible.
---
## Fast-Auth: Email onboarding
One of the first barriers that new users face when entering the world of Web3 is the need to create a crypto wallet. This generally implies the need to choose a wallet, create and store a recovery phrase, and obtain deposit funds to start using the account.
With FastAuth, users only need to provide an email address to create a NEAR account. Using the same email address the user will be able to use their account across applications and devices.
:::info
FastAuth accounts are kept safe through multi-party computation (MPC) on a decentralized network. This means that the user's private key is never stored in a single location, and thus it is never exposed to any single party.
:::
<hr subclass="subsection" />
## Relayers: Cover gas fees
Allowing users to start using a dApp without having to acquire funds is a powerful tool to increase user adoption. NEAR Protocol provides a service that allows developers to subsidize gas fees for their users.
This concept, known as "Account Abstraction" in other chains, is a **built-in feature** in NEAR. User can wrap transactions in messages known as **meta-transaction**, that any other account can relay to the network.
:::tip
In NEAR the relayers simply attach NEAR to cover gas fees, and pass the transaction to the network. There, the transaction is executed as if the **user had send it**.
:::
<hr subclass="subsection" />
## Multi-chain signatures: One account, multiple chains
Currently, users and applications are siloed in different chains. This means that a user needs to create a new account for each chain they want to use. This is not only cumbersome for the user, but also for the developer who needs to maintain different codebases for each chain.
NEAR Protocol provides a multi-chain signature service that allows users to use their NEAR Account to sign transactions in **other chains**. This means that a user can use the same account to interact with **Ethereum**, **Binance Smart Chain**, **Avalanche**, and **NEAR**.
:::info
Multi-chain signatures work by combining **smart contracts** that produce signatures, with indexers that listen for these signatures, and relayers that submit the transactions to other networks. This allows users to hold assets and use applications in **any** network, only needing to have a single NEAR account.
::: |
ICYMI: Scootering Through Web3 at NEAR APAC’s Vietnam Showcase
COMMUNITY
September 18, 2023
It was an incredible first-ever NEAR APAC, with the NEAR ecosystem scootering through Saigon over the last two days. Vietnam served as the perfect backdrop for NEAR’s inaugural showcase in Asia-Pacific, with community members, B.O.S. builders, and general Web3 enthusiasts coming together in one of the region’s most dynamic countries.
The event was spearheaded by the NEAR Vietnam Hub with support from the NEAR Foundation, exemplifying strong commitment and collaboration between local and global ecosystem stakeholders. ICYMI, here are some of the biggest sessions, announcements, and events from NEAR APAC 2023.
ICYMI, here are some of the biggest sessions, announcements, and events from NEAR APAC 2023.
Web3’s bright investment outlook in Asia-Pacific
One of the most encouraging and optimistic sessions was moderated by NEAR’s Head of Finance, David Norris, focusing on the current and future outlook of blockchain investment and development in the region. David was joined by Web3 venture veterans Riccardo Pagano from Outlier Ventures and Whiplus Wang of IVC crypto.
The trio dove into the current state of blockchain investment in APAC, and how Web3 might just ascend to becoming one of the most invested sectors in the region from a venture and infrastructure perspective. Ricardo in particular emphasized that he’s seeing a shift in perception from investors, with Web3 going from a niche technology to its own broad-based sector.
“Even though we’re technically in a bear market, investment in Web3 sub-sectors like gaming is still strong,” added Whiplus. “Games like Angry Birds and Fruit Ninja were instrumental in onboarding Web2 users onto the smartphone, for example. That’s why investment into blockchain game publishers is increasing — to find that killer game that onboards billions.”
Whiplus also likened successful blockchain startups to a bowl of Pho, with a blend of technical and business elements being integral to a delicious final dish. The panel also touched on technical nuances like ZK rollups and modular blockchains, emphasizing the need to invest in talent and innovation through initiatives like hackathons.
The session encapsulated a forward-looking perspective on Web3’s trajectory. With the bar for founder participation evidently rising, the panel stressed the importance of education in the space. There was unanimous optimism about Southeast Asia’s digital future as potentially outpacing even established tech hubs like Korea and Japan.
NEAR supports B.O.S. and blockchain builders in APAC
Tons of activity also took place in and around the Builder Stage, with the NEAR Foundation and local blockchain organizations teaming up to educate and assist developers, builders, and job seekers in advancing themselves into Web3. Cameron Dennis, CEO of Banyan, led a panel on the stage informing developers on how to upskill from Web2 to Web3.
Local developer advocates from notable projects like the Graph and Khyber network also participated in sessions, workshops, and panels on the Builder Stage designed to upskill local blockchain professionals. There was something for everyone, from a job fair for Web3 newbies to a Greenhouse area connecting founders and projects to VCs for funding.
Exploring everything from dApp architecture to tokenomics, the Builder Stage action was highlighted by an incredible Hackathon, with participants receiving guidance from experts like Oleg Fomenko, founder of SWEAT. On the final night of the festivities, NEAR co-founder Illia Polosukhin presented awards to the winners on stage in front of a packed main hall.
Pagoda takes the main stage to talk user adoption and FastAuth
Alex Chiocchi, the CPO of Pagoda, showcased FastAuth during his session titled “Unlocking the Power of B.O.S: Product Update for Founders & Developers.” FastAuth aims to redefine the user experience for developers and founders, addressing the longstanding challenges of both onboarding and retaining new Web3 users.
A primary takeaway was how FastAuth’s next release will minimize gas fees when onboarding, thus removing barriers for end-users. The introduction of multiparty compute (MPC) allows proofs from various organizations to be combined, simplifying processes like email recovery. Chiocchi emphasized the potential for users to migrate effortlessly from Web2 logins, hinting at a seamless integration between the two web generations.
Discussing the nuances of retaining users in Web3, Chiocchi stressed that coin incentives alone aren’t sufficient. The “toothbrush test” was highlighted, illustrating that many apps don’t become daily necessities for users. Trust plays a pivotal role, especially with the rise of scams in the decentralized space.
Side events and after-parties storm the streets of Saigon
NEAR APAC fully embraced the “work hard, play hard” ethos of Vietnam and Asia-Pacific. Even during the event, there were LED dancers, Manga Cosplay, and several massive DJs and party breaks. Not to mention the NEAR APAC Opening Night party at the Intercontinental Hotel, where Illia officially welcomed attendees and kicked things off in an intimate setting.
And in one of the coolest side events in recent NEAR memory, Dap Dap showcased its platform to B.O.S. enthusiasts at the My House meeting venue nestled in one of Saigon’s many cozily-lit alleyways. Dap Dap is the first commercial B.O.S. product and will act as a gateway to hundreds of chains and protocols for users from a single, user-friendly interface.
B.O.S Beats by DAO Records was the grand finale of NEAR APAC, offering a vibrant blend of hip-hop beats from prominent DJs on the decentralized record label. Held at Indika Saigon — and with pizza supplied by Pizza DAO — attendees enjoyed live performances, refreshments, and networking opportunities with the global and APAC NEAR communities.
NEAR APAC was an incredible Web3 Bánh Mì, layering an eclectic mix of speakers, insights, and events to create something completely unique. The NEAR Foundation would like to thank every cook in the kitchen, especially local partners like the NEAR Vietnam Hub who made sure that the first ever NEAR APAC had the perfect mix of sweet, sour, and spicy.
|
---
sidebar_label: "Lake Framework"
---
# NEAR Lake Framework
:::note GitHub repo
https://github.com/near/near-lake-framework/
:::
## Description
NEAR Lake Framework is an ecosystem of library companions to [NEAR Lake](/tools/realtime#near-lake-indexer). They allow you to build your own indexer that subscribes to the stream of blocks from the [NEAR Lake](/tools/realtime#near-lake-indexer) data source and create your own logic to process the NEAR Protocol data in the programming languages of your choice (at the moment, there are implementations in [Python](http://pypi.org/project/near-lake-framework), [JavaScript](https://www.npmjs.com/package/near-lake-framework), and [Rust](https://crates.io/crates/near-lake-framework)).
:::tip NEAR Lake Framework announcement
We have announced the release of NEAR Lake Framework on NEAR Governance Forum.
Please, read the post [there](https://gov.near.org/t/announcement-near-lake-framework-brand-new-word-in-indexer-building-approach/17668).
:::
## How does it compare to [NEAR Indexer Framework](near-indexer-framework.md)?
Feature | Indexer Framework | Lake Framework
------- | ----------------- | --------------
Allows to follow the blocks and transactions in the NEAR Protocol | **Yes** | **Yes**<br />(but only mainnet and testnet networks)
Decentralized | **Yes** | No<br />(Pagoda Inc dumps the blocks to AWS S3)
Reaction time (end-to-end) | [minimum 3.8s (estimated average 5-7s)](near-indexer-framework.md#limitations) | [minimum 3.9s (estimated average 6-8s)](#limitations)
Reaction time (framework overhead only) | 0.1s | 0.2-2.2s
Estimated cost of infrastructure | [$500+/mo](https://near-nodes.io/rpc/hardware-rpc) | [**$20/mo**](#what-is-the-cost-of-running-a-custom-indexer-based-on-near-lake)
Ease of maintenance | Advanced<br />(need to follow every nearcore upgrade, and sync state) | **Easy**<br />(deploy once and forget)
How long will it take to start? | days (on mainnet/testnet) | **seconds**
Ease of local development | Advanced<br />(localnet is a good option, but testing on testnet/mainnet is too heavy) | **Easy**<br />(see [tutorials](/build/data-infrastructure/lake-framework/near-lake-state-changes-indexer))
Programming languages that a custom indexer can be implemented with | Rust only | **Any**<br />(currently, helper packages are released in [Python](http://pypi.org/project/near-lake-framework), [JavaScript](https://www.npmjs.com/package/near-lake-framework), and [Rust](https://crates.io/crates/near-lake-framework))
## Limitations
Lake Framework relies on the data being dumped to AWS S3 from [NEAR Lake Indexer](https://github.com/near/near-lake-indexer) which is based on [NEAR Indexer Framework](near-indexer-framework.md). Thus, Lake Framework is centralized around AWS S3 storage and also around maintainers of NEAR Lake Indexer nodes (Pagoda Inc). This is the tradeoff you might still want to take given all the other benefits mentioned above.
Indexers based on the Lake Framework inherit [the latency characteristics of Indexer Framework](near-indexer-framework.md#limitations) and extra latency of dumping to and reading from AWS S3, which is estimated to add at least 50ms delay while writing to S3, and 50ms on polling and reading from S3 (to make the polling cost-efficient, we default to polling only every 2s, so in the worst case you may observe an additional 2-second latency). Thus, Lake Framework adds 0.1-2.1s latency on top of Indexer Framework. Yet, again, most of the latency is there due to the finalization delay and both Indexer Framework and Lake Framework add quite a minimum overhead.
## What is the cost of running a custom indexer based on NEAR Lake?
Indexers based on NEAR Lake consume 100-500MB of RAM depending on the size of the preloading queue, it does not require any storage, and it can potentially run even on Raspberry PI.
Getting the blockchain data from S3 will cost around $18.15 per month as NEAR Lake configured S3 buckets in such a way that the reader is paying the costs.
### AWS S3 cost breakdown
Assuming NEAR Protocol produces exactly 1 block per second (which is really not, the average block production time is 1.3s). A full day consists of 86400 seconds, that's the max number of blocks that can be produced.
According the [Amazon S3 prices](https://aws.amazon.com/s3/pricing/?nc1=h_ls) `list` requests are charged for $0.005 per 1000 requests and `get` is charged for $0.0004 per 1000 requests.
Calculations (assuming we are following the tip of the network all the time):
```
86400 blocks per day * 5 requests for each block / 1000 requests * $0.0004 per 1k requests = $0.173 * 30 days = $5.19
```
**Note:** 5 requests for each block means we have 4 shards (1 file for common block data and 4 separate files for each shard)
And a number of `list` requests we need to perform for 30 days:
```
86400 blocks per day / 1000 requests * $0.005 per 1k list requests = $0.432 * 30 days = $12.96
$5.19 + $12.96 = $18.15
```
Note, the price depends on the number of shards.
## Examples & Tutorials
- [`near-examples/near-lake-raw-printer`](https://github.com/near-examples/near-lake-raw-printer): simple example of a data printer built on top of NEAR Lake Framework
- [`near-examples/near-lake-accounts-watcher`](https://github.com/near-examples/near-lake-accounts-watcher): source code for a video tutorial on how to use the NEAR Lake Framework
- [`near-examples/indexer-tx-watcher-example-lake`](https://github.com/near-examples/indexer-tx-watcher-example-lake) indexer example that watches for transaction for specified accounts/contracts build on top of NEAR Lake Framework
:::note Tutorials
See [Tutorials page](/build/data-infrastructure/lake-framework/near-lake-state-changes-indexer)
:::
|
---
id: near
title: Interacting with Near
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
import {WidgetEditor} from "@site/src/components/widget-editor"
The components can use the `Near` object to interact with smart contracts in the NEAR blockchain. There are three methods:
- [`Near.view`](#nearview)
- [`Near.block`](#nearblock)
- [`Near.call`](#nearcall)
---
## Near.view
Queries a read-only method from a NEAR smart contract, returning:
- **`null`**: If the query is still being processed
- **`undefined`**: If the query is complete and no value was returned by the contract
- A **value**: If the query is complete and a value was returned by the contract
<WidgetEditor>
```js
const greeting = Near.view("hello.near-examples.testnet", "get_greeting", {});
if (greeting === null) return "Loading...";
return `The contract says: ${greeting}`;
```
</WidgetEditor>
<details markdown="1">
<summary> Parameters </summary>
| param | required | type | description |
|--------------------|--------------|-----------------|--------------------------------------------------------------------------------------------------------------------------------|
| `contractName` | **required** | string | Name of the smart contract |
| `methodName` | **required** | string | Name of the method to call |
| `args` | _optional_ | object instance | Arguments to pass to the method |
| `blockId/finality` | _optional_ | string | Block ID or finality of the transaction |
| `subscribe` | _optional_ | boolean | This feature allows users to subscribe to a query, which automatically refreshes the data for all subscribers every 5 seconds. |
</details>
:::tip
Notice that the optional parameter `subscribe` allows users to subscribe to a query, which automatically refreshes the data every 5 seconds.
:::
<hr className="subsection" />
#### Avoiding a Common Pitfall
If you want to initialize the state with the result of a `Near.view` call, be sure to check first that the value was obtained, to avoid initializing the state with `null`.
<WidgetEditor>
```js
const contractGreet = Near.view("hello.near-examples.testnet", "get_greeting", {});
// you need to first check that the value was obtained
if (contractGreet === null) return "Loading...";
const [greeting, setGreeting] = useState(contractGreet);
return `The contract says: ${greeting}`;
```
</WidgetEditor>
If you don't want to delay the render of your component, you can also use the `useEffect` hook to control the value returned by `Near.view`
<WidgetEditor>
```js
const contractGreet = Near.view('hello.near-examples.testnet', 'get_greeting');
const [greeting, setGreeting] = useState('Loading ...');
useEffect(() => {
if (contractGreet !== null) setGreeting(contractGreet);
}, [contractGreet]);
return `The contract says: ${greeting}`;
```
</WidgetEditor>
---
## Near.call
Calls a smart contract method from the blockchain. Since a transaction needs to be signed, the user must be logged in in order to make the call.
<WidgetEditor>
```js
if (!context.accountId) return "Please login...";
const onClick = () => {
Near.call(
"hello.near-examples.testnet",
"set_greeting",
{ greeting: "Hello!" }
);
};
return <>
<h5> Hello {context.accountId} </h5>
<button onClick={onClick}> Set Greeting </button>
</>;
```
</WidgetEditor>
<details markdown="1">
<summary> Parameters </summary>
| param | required | type | description |
|----------------|--------------|-----------------|-----------------------------------------------------------------------------|
| `contractName` | **required** | string | Name of the smart contract to call |
| `methodName` | **required** | string | Name of the method to call on the smart contract |
| `args` | _optional_ | object instance | Arguments to pass to the smart contract method as an object instance |
| `gas` | _optional_ | string / number | Maximum amount of gas to be used for the transaction (default 300Tg) |
| `deposit` | _optional_ | string / number | Amount of NEAR tokens to attach to the call as deposit (in yoctoNEAR units) |
</details>
:::tip
Remember that you can login using the `Login` button at the navigation bar.
:::
---
## Near.block
Queries a block from the blockchain.
<WidgetEditor height="40px">
```js
return Near.block("optimistic");
```
</WidgetEditor>
<details markdown="1">
<summary> Parameters </summary>
| param | required | type | description |
|-------------------------|------------|------|---------------------------------------------------------------------------------------------------------------------------------------------------|
| `blockHeightOrFinality` | _optional_ | any | The block height or finality level to use for the blockchain query (desired block height, or one of the following strings: `optimistic`, `final`) |
- desired block height: The height of the specific block to query, expressed as a positive integer
- `optimistic`: Uses the latest block recorded on the node that responded to your query (< 1 second delay)
- `final`: a block that has been validated on at least 66% of the nodes in the network (approx. 2s)
</details> |
---
id: data-storage
title: "Data Storage / Collections"
sidebar_label: Storage on NEAR
---
All data stored on the NEAR blockchain is done in key / value pairs. There are several collection methods in the SDKs we've created that will help you store your data on chain.
- [`near-sdk-rs`](https://github.com/near/near-sdk-rs) for [Rust](https://www.rust-lang.org/) smart contracts
- [`near-sdk-js`](https://github.com/near/near-sdk-js) for [JavaScript](https://www.javascript.com/) smart contracts
For information on storage costs, please see [ **[storage staking](/concepts/storage/storage-staking)** ].
---
## Rust Collection Types {#rust-collection-types}
[`near-sdk-rs` module documentation](https://docs.rs/near-sdk/latest/near_sdk/collections/)
| Type | Iterable | Clear All Values | Preserves Insertion Order | Range Selection |
| ------------------------------------------------------------- | :------: | :--------------: | :-----------------------: | :-------------: |
| [`Vector`](/concepts/storage/data-storage#vector) | ✅ | ✅ | ✅ | ✅ |
| [`LookupSet`](/concepts/storage/data-storage#lookupset) | | | | |
| [`UnorderedSet`](/concepts/storage/data-storage#unorderedset) | ✅ | ✅ | | ✅ |
| [`LookupMap`](/concepts/storage/data-storage#lookupmap) | | | | |
| [`UnorderedMap`](/concepts/storage/data-storage#unorderedmap) | ✅ | ✅ | | ✅ |
| [`TreeMap`](/concepts/storage/data-storage#treemap) | ✅ | ✅ | | |
---
### Big-O Notation {#big-o-notation-1}
> The [Big-O notation](https://en.wikipedia.org/wiki/Big_O_notation) values in the chart below describe the [time complexity](https://en.wikipedia.org/wiki/Time_complexity) of the various collection methods found in `near-sdk-rs`. These method complexities correlate with [gas](/concepts/protocol/gas) consumption on NEAR, helping you decide which collection to utilize in your project. There are three types found in our collection methods:
- O(1) - _[constant](https://en.wikipedia.org/wiki/Time_complexity#Constant_time)_
- O(n) - _[linear](https://en.wikipedia.org/wiki/Time_complexity#Linear_time)_
- O(log n) - _[logarithmic](https://en.wikipedia.org/wiki/Time_complexity#Logarithmic_time)_
| Type | Access | Insert | Delete | Search | Traverse | Clear |
| ------------------------------------------------------------- | :------: | :------: | :------: | :------: | :------: | :---: |
| [`Vector`](/concepts/storage/data-storage#vector) | O(1) | O(1)\* | O(1)\*\* | O(n) | O(n) | O(n) |
| [`LookupSet`](/concepts/storage/data-storage#lookupset) | O(1) | O(1) | O(1) | O(1) | N/A | N/A |
| [`UnorderedSet`](/concepts/storage/data-storage#unorderedset) | O(1) | O(1) | O(1) | O(1) | O(n) | O(n) |
| [`LookupMap`](/concepts/storage/data-storage#lookupmap) | O(1) | O(1) | O(1) | O(1) | N/A | N/A |
| [`UnorderedMap`](/concepts/storage/data-storage#unorderedmap) | O(1) | O(1) | O(1) | O(1) | O(n) | O(n) |
| [`TreeMap`](/concepts/storage/data-storage#treemap) | O(1) | O(log n) | O(log n) | O(log n) | O(n) | O(n) |
_\* - to insert at the end of the vector using `push_back` (or `push_front` for deque)_
_\*\* - to delete from the end of the vector using `pop` (or `pop_front` for deque), or delete using `swap_remove` which swaps the element with the last element of the vector and then removes it._
---
### Gas Consumption Examples {#gas-consumption-examples-1}
> The examples below show differences in gas burnt storing and retrieving key/value pairs using the above methods. Please note that the gas cost of spinning up the runtime environment on chain has been deducted to show just data read/writes.
>
> You can reproduce this and test out your own data set by visiting [collection-examples-rs](https://github.com/near-examples/collection-examples-rs).


---
### `Vector` {#vector}
> Implements a [vector](https://en.wikipedia.org/wiki/Array_data_structure) / persistent array.
>
> - can iterate using index
> - Uses the following map: index -> element.
[ [SDK source](https://github.com/near/near-sdk-rs/blob/master/near-sdk/src/collections/vector.rs) ]
[ [Implementation](https://docs.rs/near-sdk/latest/near_sdk/collections/vector/struct.Vector.html) ]
---
### `LookupSet` {#lookupset}
> Implements a persistent set _without_ iterators.
>
> - can not iterate over keys
> - more efficient in reads / writes
[ [SDK source](https://github.com/near/near-sdk-rs/blob/master/near-sdk/src/collections/lookup_set.rs) ]
[ [Implementation](https://docs.rs/near-sdk/latest/near_sdk/collections/struct.LookupSet.html) ]
---
### `UnorderedSet` {#unorderedset}
> Implements a persistent set _with_ iterators for keys, values, and entries.
[ [SDK source](https://github.com/near/near-sdk-rs/blob/master/near-sdk/src/collections/unordered_set.rs) ]
[ [Implementation Docs](https://docs.rs/near-sdk/latest/near_sdk/collections/struct.UnorderedSet.html) ]
---
### `LookupMap` {#lookupmap}
> Implements a persistent map.
>
> - can not iterate over keys
> - does not preserve order when removing and adding values
> - efficient in number of reads and writes
- To add data:
```rust
pub fn add_lookup_map(&mut self, key: String, value: String) {
self.lookup_map.insert(&key, &value);
}
```
- To get data:
```rust
pub fn get_lookup_map(&self, key: String) -> String {
match self.lookup_map.get(&key) {
Some(value) => {
let log_message = format!("Value from LookupMap is {:?}", value.clone());
env::log(log_message.as_bytes());
value
},
None => "not found".to_string()
}
}
```
[ [SDK source](https://github.com/near/near-sdk-rs/blob/master/near-sdk/src/collections/lookup_map.rs) ]
[ [Implementation](https://docs.rs/near-sdk/latest/near_sdk/collections/struct.LookupMap.html) ]
---
### `UnorderedMap` {#unorderedmap}
> Implements an unordered map.
>
> - iterable
> - does not preserve order when removing and adding values
> - is able to clear all values
- To add data:
```rust
pub fn add_unordered_map(&mut self, key: String, value: String) {
self.unordered_map.insert(&key, &value);
}
```
- To get data:
```rust
pub fn get_unordered_map(&self, key: String) -> String {
match self.unordered_map.get(&key) {
Some(value) => {
let log_message = format!("Value from UnorderedMap is {:?}", value.clone());
env::log(log_message.as_bytes());
value
},
// None => "Didn't find that key.".to_string()
None => "not found".to_string()
}
}
```
[ [SDK source](https://github.com/near/near-sdk-rs/tree/master/near-sdk/src/collections/unordered_map) ]
[ [Implementation](https://docs.rs/near-sdk/latest/near_sdk/collections/unordered_map/struct.UnorderedMap.html) ]
---
### `TreeMap` {#treemap}
> Implements a Tree Map based on [AVL-tree](https://en.wikipedia.org/wiki/AVL_tree).
>
> - iterable
> - preserves order
> - able to clear all values
> - self balancing
- To add data:
```rust
pub fn add_tree_map(&mut self, key: String, value: String) {
self.tree_map.insert(&key, &value);
}
```
- To get data:
```rust
pub fn get_tree_map(&self, key: String) -> String {
match self.tree_map.get(&key) {
Some(value) => {
let log_message = format!("Value from TreeMap is {:?}", value.clone());
env::log(log_message.as_bytes());
// Since we found it, return it (note implicit return)
value
},
// did not find the entry
// note: curly brackets after arrow are optional in simple cases, like other languages
None => "not found".to_string()
}
}
```
[ [SDK source](https://github.com/near/near-sdk-rs/blob/master/near-sdk/src/collections/tree_map.rs) ]
[ [Implementation](https://docs.rs/near-sdk/latest/near_sdk/collections/struct.TreeMap.html) ]
---
## Storage Constraints on NEAR
For storing data on-chain it’s important to keep in mind the following:
- Can add up in storage staking costs
- There is a 4mb limit on how much you can upload at once
Let’s say for example, someone wants to put an NFT purely on-chain (rather than IPFS or some other decentralized storage solution) you’ll have almost an unlimited amount of storage but will have to pay 1 $NEAR per 100kb of storage used (see Storage Staking).
Users will be limited to 4MB per contract call upload due to MAX_GAS constraints. The maximum amount of gas one can attach to a given functionCall is 300TGas.
|
Randomness in blockchain protocols
DEVELOPERS
August 9, 2019
We have published today a short paper that describes the randomness beacon that is used in NEAR Protocol. In this accompanying blog post we discuss why randomness is important, why it is hard, and how it is addressed in other protocols.
Many modern blockchain protocols, including NEAR, rely on a source of randomness for selecting participants that carry out certain actions in the protocol. If a malicious actor can influence such source of randomness, they can increase their chances of being selected, and possibly compromise the security of the protocol.
Distributed randomness is also a crucial building block for many distributed applications built on the blockchain. For example, a smart contract that accepts a bet from a participant, and pays out twice the amount with 49% and nothing with 51% assumes that it can get an unbiasable random number. If a malicious actor can influence or predict the random number, they can increase their chance to get the payout in the smart contract, and deplete it.
When we design a distributed randomness algorithm, we want it to have three properties:
It needs to be unbiasable. In other words, no participant shall be able to influence in any way the outcome of the random generator.
It needs to be unpredictable. In other words, no participant shall be able to predict what number will be generated (or reason about any properties of it) before it is generated.
The protocol needs to tolerate some percentage of actors that go offline or try to intentionally stall the protocol.
In this article we will cover the basics of distributed random beacons, discuss why simple approaches do not work. Finally, we will cover the approaches used by DFinity, Ethereum Serenity, and NEAR and discuss their advantages and disadvantages.
RANDAO
RANDAO is a very simple, and thus quite commonly used, approach to randomness. The general idea is that the participants of the network first all privately choose a pseudo-random number, submit a commitment to such privately chosen number, all agree on some set of commitments using some consensus algorithm, then all reveal their chosen numbers, reach a consensus on the revealed numbers, and have the XOR of the revealed numbers to be the output of the protocol.
It is unpredictable, and has the same liveness as the underlying consensus protocol, but is biasable. Specifically, a malicious actor can observe the network once others start to reveal their numbers, and choose to reveal or not to reveal their number based on XOR of the numbers observed so far. This allows a single malicious actor to have one bit of influence on the output, and a malicious actor controlling multiple participants have as many bits of influence as the number of participants they are controlling.
RANDAO + VDFs
To make RANDAO unbiasable, one approach would be to make the output not just XOR, but something that takes more time to execute than the allocated time to reveal the numbers. If the computation of the final output takes longer than the reveal phase, the malicious actor cannot predict the effect of them revealing or not revealing their number, and thus cannot influence the result.
While we want the function that computes the randomness to take a long time for the participants that generate the random number, we want the users of the random number to not have to execute the same expensive function again. Thus, they need to somehow be able to quickly verify that the random number was generated properly without redoing the expensive computation.
Such a function that takes a long time to compute, is fast to verify the computation, and has a unique output for each input is called a verifiable delay function (VDF for short) and it turns out that designing one is extremely complex. There’ve been multiple breakthroughs recently, namely this one and this one that made it possible, and Ethereum presently plans to use RANDAO with VDF as their randomness beacon. Besides the fact that this approach is unpredictable and unbiasable, it has an extra advantage that is has liveness even if only two participants are online (assuming the underlying consensus protocol has liveness with so few participants).
The biggest challenge with this approach is that the VDF needs to be configured in such a way that even a participant with very expensive specialized hardware cannot compute the VDF before the reveal phase ends, and ideally have some meaningful safety margin, say 10x. The figure below shows an attack by a participant that has a specialized ASIC that allows them to run the VDF faster than the time allocated to reveal RANDAO commitments. Such a participant can still compute the final output with and without their share, and choose to reveal or not to reveal based on those outputs:
For the family of VDFs linked above a specialized ASIC can be 100+ times faster than conventional hardware. Thus, if the reveal phase lasts 10 seconds, the VDF computed on such an ASIC must take longer than 100 seconds to have 10x safety margin, and thus the same VDF computed on the conventional hardware needs to take 100 x 100 seconds = ~3 hours.
The way Ethereum Foundation plans to address it is to design its own ASICs and make them publicly available for free. Once this happens, all other protocols can take advantage of the technology, but until then the RANDAO + VDFs approach is not as viable for protocols that cannot invest in designing their own ASICs.
This website accumulates lots of articles, videos and other information on VDFs.
Threshold Signatures
Another approach to randomness, pioneered by Dfinity, is to use so-called threshold BLS signatures. (Fun fact, Dfinity employs Ben Lynn, who is the L in BLS).
BLS signatures is a construction that allows multiple parties to create a single signature on a message, which is often used to save space and bandwidth by not requiring sending around multiple signatures. A common usage for BLS signatures in blockchains is signing blocks in BFT protocols. Say 100 participants create blocks, and a block is considered final if 67 of them sign on it. They can all submit their parts of the BLS signatures, and then use some consensus algorithm to agree on 67 of them and then aggregate them into a single BLS signature. Any 67 parts can be used to create an accumulated signature, however the resulting signature will not be the same depending on what 67 signatures were aggregated.
Turns out that if the private keys that the participants use are generated in a particular fashion, then no matter what 67 (or more, but not less) signatures are aggregated, the resulting multisignature will be the same. This can be used as a source of randomness: the participants first agree on some message that they will sign (it could be an output of RANDAO, or just the hash of the last block, doesn’t really matter for as long as it is different every time and is agreed upon), and create a multisignature on it. Until 67 participants provide their parts, the output is unpredictable, but even before the first part is provided the output is already predetermined and cannot be influenced by any participant.
This approach to randomness is completely unbiasable and unpredictable, and is live for as long as 2/3 of participants are online (though can be configured for any threshold). While ⅓ offline or misbehaving participants can stall the algorithm, it takes at least ⅔ participants to cooperate to influence the output.
There’s a caveat, however. Above, I mentioned that the private keys for this scheme need to be generated in a particular fashion. The procedure for the key generation, called Distributed Key Generation, or DKG for short, is extremely complex and is an area of active research. In one of the recent talks, Dfinity presented a very complex approach which involved zk-SNARKs, a very sophisticated and not time tested cryptographic construction, as one of the steps. Generally, the research on threshold signatures and DKGs in particular is not in a state where it can be easily applied in practice.
RandShare
The NEAR approach is influenced by yet another algorithm called RandShare. RandShare is an unbiasable and unpredictable protocol that can tolerate up to ⅓ of the actors being malicious. It is relatively slow, and the paper linked also describes two ways to speed it up, called RandHound and RandHerd, but unlike RandShare itself RandHound and RandHerd are relatively complex, while we wish the protocol to be extremely simple.
The general problems with RandShare besides the large number of messages exchanged (the participants together will exchange O(n^3) messages), is the fact that while ⅓ is a meaningful threshold for liveness in practice, it is low for the ability to influence the output. There are several reasons for it:
The benefit from influencing the output can significantly outweigh the benefit of stalling randomness.
If a participant controls more than ⅓ of participants in RandShare and uses this to influence the output, it leaves no trace. Thus a malicious actor can do it without ever being revealed. Stalling a consensus is naturally visible.
The situations in which someone controls ⅓ of hashpower / stake are not impossible, and given (1) and (2) above someone having such control is unlikely to attempt to stall the randomness, but can and likely will attempt to influence it.
NEAR Approach
NEAR Approach is described in a paper we recently published. It is unbiasable and unpredictable, and can tolerate up to ⅓ malicious actors for liveness, i.e. if someone controls ⅓ or more participants, they can stall the protocol.
However, unlike RandShare, it tolerates up to ⅔ malicious actors before one can influence the output. This is significantly better threshold for practical applications.
The core idea of the protocol is the following (for simplicity assuming there are exactly 100 participants):
Each participant comes up with their part of the output, splits it into 67 parts, erasure codes it to obtain 100 shares such that any 67 are enough to reconstruct the output, assigns each of the 100 shares to one of the participants and encodes it with the public key of such participant. They then share all the encoded shares.
The participants use some consensus (e.g. Tendermint) to agree on such encoded sets from exactly 67 participants.
Once the consensus is reached, each participant takes the encoded shares in each of the 67 sets published this way that is encoded with their public key, then decodes all such shares and publishes all such decoded shares at once.
Once at least 67 participants did the step (3), all the agreed upon sets can be fully decoded and reconstructed, and the final number can be obtained as an XOR of the initial parts the participants came up with in (1).
The idea why this protocol is unbiasable and unpredictable is similar to that of RandShare and threshold signatures: the final output is decided once the consensus is reached, but is not known to anyone until ⅔ of the participants decrypt shares encrypted with their public key.
Handling all the corner cases and possible malicious behaviors make it slightly more complicated (for example, the participants need to handle the situation when someone in step (1) created an invalid erasure code), but overall the entire protocol is very simple, such that the entire paper that describes it with all the proofs, the corresponding cryptographic primitives and references is just 7 pages long. Make sure to check it out if you want to read a more formal description of the algorithm, or the analysis of its liveness and resistance to influence.
Similar idea that leverages erasure codes is already used in the existing infrastructure of NEAR, in which the block producers in a particular epoch create so-called chunks that contain all the transaction for a particular shard, and distribute erasure-coded versions of such chunks with merkle proofs to other block producers to ensure data availability (see section 3.4 of our sharding paper here).
Outro
This write-up is part of an ongoing effort to create high quality technical content about blockchain protocols and related topics. We also run a video series in which we talk to the founders and core developers of many protocols, we have episodes withEthereum Serenity,Cosmos,Polkadot,Ontology,QuarkChain and many other protocols. All the episodes are conveniently assembled into a playlist here.
If you are interested to learn more about technology behind NEAR Protocol, make sure to check out the above-mentioned sharding paper. NEAR is one of the very few protocols that addresses the state validity and data availability problems in sharding, and the paper contains great overview of sharding in general and its challenges besides presenting our approach.
While scalability is a big concern for blockchains today, a bigger concern is usability. We invest a lot of effort into building the most usable blockchain. We published an overview of the challenges that the blockchain protocols face today with usability, and how they can be addressed here.
Follow us on Twitter and join our Discord chat where we discuss all the topics related to tech, economics, governance and other aspects of building a protocol. |
---
id: core
title: Core
sidebar_label: Core
---
import {Github} from "@site/src/components/codetabs"
In this tutorial you'll learn how to implement the [core standards](https://nomicon.io/Standards/Tokens/NonFungibleToken/Core) into your smart contract. If you're joining us for the first time, feel free to clone [this repo](https://github.com/near-examples/nft-tutorial) and checkout the `3.enumeration` branch to follow along.
```bash
git checkout 3.enumeration
```
:::tip
If you wish to see the finished code for this _Core_ tutorial, you can find it on the `4.core` branch.
:::
## Introduction {#introduction}
Up until this point, you've created a simple NFT smart contract that allows users to mint tokens and view information using the [enumeration standards](https://nomicon.io/Standards/Tokens/NonFungibleToken/Enumeration). Today, you'll expand your smart contract to allow for users to not only mint tokens, but transfer them as well.
As we did in the [minting tutorial](/tutorials/nfts/js/minting), let's break down the problem into multiple subtasks to make our lives easier. When a token is minted, information is stored in 3 places:
- **tokensPerOwner**: set of tokens for each account.
- **tokensById**: maps a token ID to a `Token` object.
- **tokenMetadataById**: maps a token ID to its metadata.
Let's now consider the following scenario. If Benji owns token A and wants to transfer it to Mike as a birthday gift, what should happen? First of all, token A should be removed from Benji's set of tokens and added to Mike's set of tokens.
If that's the only logic you implement, you'll run into some problems. If you were to do a `view` call to query for information about that token after it's been transferred to Mike, it would still say that Benji is the owner.
This is because the contract is still mapping the token ID to the old `Token` object that contains the `owner_id` field set to Benji's account ID. You still have to change the `tokensById` data structure so that the token ID maps to a new `Token` object which has Mike as the owner.
With that being said, the final process for when an owner transfers a token to a receiver should be the following:
- Remove the token from the owner's set.
- Add the token to the receiver's set.
- Map a token ID to a new `Token` object containing the correct owner.
:::note
You might be curious as to why we don't edit the `tokenMetadataById` field. This is because no matter who owns the token, the token ID will always map to the same metadata. The metadata should never change and so we can just leave it alone.
:::
At this point, you're ready to move on and make the necessary modifications to your smart contract.
## Modifications to the contract
Let's start our journey in the `nft-contract/src/nft_core.ts` file.
### Transfer function {#transfer-function}
You'll start by implementing the `nft_transfer` logic. This function will transfer the specified `token_id` to the `receiver_id` with an optional `memo` such as `"Happy Birthday Mike!"`. The core logic will be found in the `internalNftTransfer` function.
<Github language="js" start="37" end="64" url="https://github.com/near-examples/nft-tutorial-js/blob/4.core/src/nft-contract/nft_core.ts" />
There are a couple things to notice here. Firstly, we've introduced a new function called `assertOneYocto()`. This method will ensure that the user has attached exactly one yoctoNEAR to the call. If a function requires a deposit, you need a full access key to sign that transaction. By adding the one yoctoNEAR deposit requirement, you're essentially forcing the user to sign the transaction with a full access key.
Since the transfer function is potentially transferring very valuable assets, you'll want to make sure that whoever is calling the function has a full access key.
Secondly, we've introduced an `internalTransfer` method. This will perform all the logic necessary to transfer an NFT.
### Internal helper functions
Let's quickly move over to the `nft-contract/src/internal.ts` file so that you can implement the `assertOneYocto()` and `internalTransfer` methods.
Let's start with the easier one, `assertOneYocto()`.
#### assertOneYocto
<Github language="js" start="38" end="41" url="https://github.com/near-examples/nft-tutorial-js/blob/4.core/src/nft-contract/internal.ts" />
#### internal_transfer
It's now time to implement the `internalTransfer` function which is the core of this tutorial. This function will take the following parameters:
- **senderId**: the account that's attempting to transfer the token.
- **receiverId**: the account that's receiving the token.
- **tokenId**: the token ID being transferred.
- **memo**: an optional memo to include.
The first thing you'll want to do is to make sure that the sender is authorized to transfer the token. In this case, you'll just make sure that the sender is the owner of the token. You'll do that by getting the `Token` object using the `token_id` and making sure that the sender is equal to the token's `owner_id`.
Second, you'll remove the token ID from the sender's list and add the token ID to the receiver's list of tokens. Finally, you'll create a new `Token` object with the receiver as the owner and remap the token ID to that newly created object.
<Github language="js" start="80" end="114" url="https://github.com/near-examples/nft-tutorial-js/blob/4.core/src/nft-contract/internal.ts" />
You've previously implemented functionality for adding a token ID to an owner's set but you haven't created the functionality for removing a token ID from an owner's set. Let's do that now by created a new function called `internalRemoveTokenFromOwner` which we'll place right above our `internalTransfer` and below the `internalAddTokenToOwner` function.
In the remove function, you'll get the set of tokens for a given account ID and then remove the passed in token ID. If the account's set is empty after the removal, you'll simply remove the account from the `tokensPerOwner` data structure.
<Github language="js" start="60" end="78" url="https://github.com/near-examples/nft-tutorial-js/blob/4.core/src/nft-contract/internal.ts" />
With these internal functions complete, the logic for transferring NFTs is finished. It's now time to move on and implement `nft_transfer_call`, one of the most integral yet complicated functions of the standard.
### Transfer call function {#transfer-call-function}
Let's consider the following scenario. An account wants to transfer an NFT to a smart contract for performing a service. The traditional approach would be to use an approval management system, where the receiving contract is granted the ability to transfer the NFT to themselves after completion. You'll learn more about the approval management system in the [approvals section](/tutorials/nfts/js/approvals) of the tutorial series.
This allowance workflow takes multiple transactions. If we introduce a “transfer and call” workflow baked into a single transaction, the process can be greatly improved.
For this reason, we have a function `nft_transfer_call` which will transfer an NFT to a receiver and also call a method on the receiver's contract all in the same transaction.
<Github language="js" start="66" end="125" url="https://github.com/near-examples/nft-tutorial-js/blob/4.core/src/nft-contract/nft_core.ts" />
The function will first assert that the caller attached exactly 1 yocto for security purposes. It will then transfer the NFT using `internalTransfer` and start the cross contract call. It will call the method `nft_on_transfer` on the `receiver_id`'s contract which returns a promise. After the promise finishes executing, the function `nft_resolve_transfer` is called. This is a very common workflow when dealing with cross contract calls. You first initiate the call and wait for it to finish executing. You then invoke a function that resolves the result of the promise and act accordingly.
In our case, when calling `nft_on_transfer`, that function will return whether or not you should return the NFT to it's original owner in the form of a boolean. This is logic will be executed in the `internalResolveTransfer` function.
<Github language="js" start="127" end="187" url="https://github.com/near-examples/nft-tutorial-js/blob/4.core/src/nft-contract/nft_core.ts" />
If `nft_on_transfer` returned true, you should send the token back to it's original owner. On the contrary, if false was returned, no extra logic is needed. As for the return value of `nft_resolve_transfer`, the standard dictates that the function should return a boolean indicating whether or not the receiver successfully received the token or not.
This means that if `nft_on_transfer` returned true, you should return false. This is because if the token is being returned to its original owner. The `receiver_id` didn't successfully receive the token in the end. On the contrary, if `nft_on_transfer` returned false, you should return true since we don't need to return the token and thus the `receiver_id` successfully owns the token.
With that finished, you've now successfully added the necessary logic to allow users to transfer NFTs. It's now time to deploy and do some testing.
## Redeploying the contract {#redeploying-contract}
Using the build script, build and deploy the contract as you did in the previous tutorials:
```bash
yarn build && near deploy --wasmFile build/nft.wasm --accountId $NFT_CONTRACT_ID
```
This should output a warning saying that the account has a deployed contract and will ask if you'd like to proceed. Simply type `y` and hit enter.
```
This account already has a deployed contract [ AKJK7sCysrWrFZ976YVBnm6yzmJuKLzdAyssfzK9yLsa ]. Do you want to proceed? (y/n)
```
:::tip
If you haven't completed the previous tutorials and are just following along with this one, simply create an account and login with your CLI using `near login`. You can then export an environment variable `export NFT_CONTRACT_ID=YOUR_ACCOUNT_ID_HERE`.
:::
## Testing the new changes {#testing-changes}
Now that you've deployed a patch fix to the contract, it's time to move onto testing. Using the previous NFT contract where you had minted a token to yourself, you can test the `nft_transfer` method. If you transfer the NFT, it should be removed from your account's collectibles displayed in the wallet. In addition, if you query any of the enumeration functions, it should show that you are no longer the owner.
Let's test this out by transferring an NFT to the account `benjiman.testnet` and seeing if the NFT is no longer owned by you.
### Testing the transfer function
:::note
This means that the NFT won't be recoverable unless the account `benjiman.testnet` transfers it back to you. If you don't want your NFT lost, make a new account and transfer the token to that account instead.
:::
If you run the following command, it will transfer the token `"token-1"` to the account `benjiman.testnet` with the memo `"Go Team :)"`. Take note that you're also attaching exactly 1 yoctoNEAR by using the `--depositYocto` flag.
:::tip
If you used a different token ID in the previous tutorials, replace `token-1` with your token ID.
:::
```bash
near call $NFT_CONTRACT_ID nft_transfer '{"receiver_id": "benjiman.testnet", "token_id": "token-1", "memo": "Go Team :)"}' --accountId $NFT_CONTRACT_ID --depositYocto 1
```
If you now query for all the tokens owned by your account, that token should be missing. Similarly, if you query for the list of tokens owned by `benjiman.testnet`, that account should now own your NFT.
### Testing the transfer call function
Now that you've tested the `nft_transfer` function, it's time to test the `nft_transfer_call` function. If you try to transfer an NFT to a receiver that does **not** implement the `nft_on_transfer` function, the contract will panic and the NFT will **not** be transferred. Let's test this functionality below.
First mint a new NFT that will be used to test the transfer call functionality.
```bash
near call $NFT_CONTRACT_ID nft_mint '{"token_id": "token-2", "metadata": {"title": "NFT Tutorial Token", "description": "Testing the transfer call function", "media": "https://bafybeiftczwrtyr3k7a2k4vutd3amkwsmaqyhrdzlhvpt33dyjivufqusq.ipfs.dweb.link/goteam-gif.gif"}, "receiver_id": "'$NFT_CONTRACT_ID'"}' --accountId $NFT_CONTRACT_ID --amount 0.1
```
Now that you've minted the token, you can try to transfer the NFT to the account `no-contract.testnet` which as the name suggests, doesn't have a contract. This means that the receiver doesn't implement the `nft_on_transfer` function and the NFT should remain yours after the transaction is complete.
```bash
near call $NFT_CONTRACT_ID nft_transfer_call '{"receiver_id": "no-contract.testnet", "token_id": "token-2", "msg": "foo"}' --accountId $NFT_CONTRACT_ID --depositYocto 1 --gas 200000000000000
```
If you query for your tokens, you should still have `token-2` and at this point, you're finished!
## Conclusion
In this tutorial, you learned how to expand an NFT contract past the minting functionality and you added ways for users to transfer NFTs. You [broke down](#introduction) the problem into smaller, more digestible subtasks and took that information and implemented both the [NFT transfer](#transfer-function) and [NFT transfer call](#transfer-call-function) functions. In addition, you deployed another [patch fix](#redeploying-contract) to your smart contract and [tested](#testing-changes) the transfer functionality.
In the [next tutorial](/tutorials/nfts/js/approvals), you'll learn about the approval management system and how you can approve others to transfer tokens on your behalf.
:::note Versioning for this article
At the time of this writing, this example works with the following versions:
- near-cli: `3.0.0`
- NFT standard: [NEP171](https://nomicon.io/Standards/Tokens/NonFungibleToken/Core), version `1.0.0`
- Enumeration standard: [NEP181](https://nomicon.io/Standards/Tokens/NonFungibleToken/Enumeration), version `1.0.0`
:::
|
NEAR Community in Focus: Decentralized Community Building on NEAR
COMMUNITY
June 16, 2022
When Matt Aaron, project lead at DeFi and NFT analytics app UniWhales, saw the explosion of interest in the NEAR protocol, he also saw a gap in the market: an app that monitored its blockchain.
The idea was to create a platform that provided real-time data for NEAR wallet activity, just like the platform he set-up to track Ethereum movements. After launching this week, the NEAR tracker bot is still a work in progress—but so far, so good.
“It was good vibes from the beginning,” says Matt. “As an analytics provider trying to tell the story of what’s going on on all these blockchains, we have to do our best to anticipate additional Layer 1s besides Ethereum where we think there can be meaningful activity our users would like to track.”
“We’re getting all the help we need to get off to a good start,”he adds. “Community members and 4NTS helped connect me to the NEAR ecosystem and get things off to a smooth start.”
Building Web3 products is different from building for Web2. Why? Because Web3 is decentralized, and users have—or at least are supposed to have—ownership. With no centralized authority making all the decisions singlehandedly, it is up to everyone to push things forward.
The importance of community in the Web3 world
For a project to be successful, those working on a protocol need to work in harmony and help newcomers. This can only be achieved with a strong community.
A recurring theme with those building NEAR products is getting a warm welcome from seasoned users of the blockchain, and the support needed to get off to a good start. “The people working on NEAR projects definitely want to help—especially newcomers,” adds Matt.
Community definitely exists in Web2. People interact on platforms like Reddit and writers can freely add to the world’s most popular websites, like Wikipedia.
But Web3 projects break down barriers, and users are much closer to the tools they use. People can have more control and say over how a project is built; there is no centralized authority (or, at least, there shouldn’t be—not for long, anyway); members can be rewarded for what they contribute.
In the Web3 world, communities are much more active and engaged (think DAOs and governance tokens.) And building a big, healthy community is key to a project’s success. The more people who believe in the project and are actively involved, the more likely it is to succeed.
How NEAR’s blockchain was built for communities
Jordan Gray, who is part of the TenK DAO and launched the NEAR Misfits NFT collection, says that building a community on NEAR is, for the most part, a smooth process—even for those moving from Web2 to Web3. This is in part due to NEAR’s unique and fool-proof architecture.
An example? NEAR’s web wallet. Getting started with it is far easier than getting started with other popular Web3 wallets, like MetaMask, says Jordan. Users get a human readable name that must be correctly entered to send money. And there are no lost funds on the NEAR blockchain. This is a feature many new users praise when entering Web3.
It’s one of the things that attracted Jordan to build upon blockchain in the first place.
“The fact that when new users had their wallets, there was a human readable name—it wasn’t a long hexadecimal code—was just very friendly in that regard,” he says. “It’s really nice and easy for new users coming from the Web2 space, they find it pretty natural and it makes sense to them.”
Naksh, an NFT marketplace built on NEAR that puts traditional art on the blockchain, has gained traction in India’s art world. Naksh co-founder Sri Lakshmi says that NEAR’s impressive architecture—the wallet in particular—has helped get people using the marketplace, in turn helping to create a buzzing community.
“I think it’s pretty simple,” she says. “I have taught my artists how to open their wallets and they did not find it that difficult being completely new to this.”
“Since all of the artists in our platform are completely new to the Web3 ecosystem and it was a first time experience for them, creating a wallet itself wasn’t much of a hassle for them,” she adds. “Including some of the deaf and dumb artists.”
NEAR’s community is already bustling, and this makes building new communities easier. With a host of guilds and DAOs already in place to help new projects launch, things go more smoothly.
“NEAR’s community thrives on cross collaborating and helping each other out,” says Sri. “We have received a lot of help from guilds and other communities on NEAR in getting more people interested in Naksh through the various giveaways, Twitter spaces, video interviews, and articles.”
A shared vision is also what keeps a decentralized community strong. And NEAR’s community shares one thing in common: a focus on building quality products.
“The kind of people who end up on NEAR are very collaborative and cooperative and want to help others,” adds Jordan. “Quality attracts quality.”
The strength of community during a bear market
NEAR Misfits co-creator Alejandro Betancourt, who does business development for the liquid staking app Meta Pool, finds strength in community. He says the NEAR community’s resilience during hard times is evidence of a solid community and project.
If anything, more people are noticing the NEAR ecosystem. Launched during the last crypto winter in 2020, it’s still going strong during today’s bear market, with more builders jumping aboard daily.
“There’s definitely a lot in the crypto culture that can be lost because everyone is just too obsessed with price or the herd mentality is too strong, so no one has the time or audacity to look into new things,” says Betancourt. “We launched during the bear market and now it’s a bear market again. The builder ethos and mentality keeps people motivated in the long-term.”
It’s a bit quiet, there is less noise, and it makes it less pump and dumpy,” he adds. “Not everything is shiny, so people get a bit more critical of projects. So when people spend time looking into NEAR technology and the community, it definitely rises to the top in ways that other projects may have been attractive when everything was pumping but are now suddenly a bit more questionable.”
This is what makes NEAR a solid project. A strong community that keeps building, growing and helping others—even when things in the wider ecosystem have quieted down. |
```bash
near view token.v2.ref-finance.near ft_metadata
```
<details>
<summary>Example response</summary>
<p>
```bash
{
spec: "ft-1.0.0",
name: "Ref Finance Token",
symbol: "REF",
icon: "data:image/svg+xml,%3Csvg xmlns='http://www.w3.org/2000/svg' viewBox='16 24 248 248' style='background: %23000'%3E%3Cpath d='M164,164v52h52Zm-45-45,20.4,20.4,20.6-20.6V81H119Zm0,18.39V216h41V137.19l-20.6,20.6ZM166.5,81H164v33.81l26.16-26.17A40.29,40.29,0,0,0,166.5,81ZM72,153.19V216h43V133.4l-11.6-11.61Zm0-18.38,31.4-31.4L115,115V81H72ZM207,121.5h0a40.29,40.29,0,0,0-7.64-23.66L164,133.19V162h2.5A40.5,40.5,0,0,0,207,121.5Z' fill='%23fff'/%3E%3Cpath d='M189 72l27 27V72h-27z' fill='%2300c08b'/%3E%3C/svg%3E%0A",
reference: null,
reference_hash: null,
decimals: 18
}
```
</p>
</details> |
---
id: onboarding-checklisk
title: Project Onboarding Checklist
sidebar_label: Project Onboarding Checklist
sidebar_position: 4
---
# NEAR Project Onboarding Checklist
---
Welcome to the NEAR ecosystem! Here are some concise and helpful guidelines to effectively onboard into the NEAR ecosystem.
- [ ] If you are interested in exploring funding to build on NEAR, there are a variety of funding tracks available depending on what you’re building - learn about them on the [Ecosystem Funding](https://near.org/ecosystem/get-funding/) page of the main near.org site to find the right one pertaining to your project
- [ ] Submit your project to [AwesomeNEAR](https://awesomenear.com/) by simply getting the details into this [form](https://awesomenear.com/getstarted)
- [ ] Get involved in the community
* NEAR Official [Discord Server](https://discord.gg/qmRAacr6ze)
* NEAR [Forum](https://gov.near.org/)
* NEAR Official [Telegram](https://t.me/cryptonear)
- [ ] If you are planning on fundraising, please submit applicable info [here](https://nearprotocol1001.typeform.com/nearvcnetwork) to get into our VC network. Submitting this will be followed up with an email to schedule a call with our head of investor relations, review your deck, and position your project in front of VCs. **This will not guarentee funding from investors/VC's.**
- [ ] For support around technical aspects:
* Daily DevRel [Office Hours](https://near.org/developers/get-help/office-hours/)
* Dev Support Discord [channel](https://discord.gg/9ezRXvQ3EG)
* Infrastructure & Tools [available](development/tools-infrastructure.md) on NEAR
- [ ] Discover talent to fill open roles and needs within your team
* NEAR Ecosystem careers portal: [here](https://careers.near.org/jobs)
* Beexperience: [here](https://beexperience.io/)
* Discover NEAR University talent: [here](https://discord.gg/k4pxafjMWA)
- [ ] Check out the available marketing & growth [resources](https://wiki.near.org/support/growth) in the NEAR ecosystem
- [ ] Don’t forget to check out the NEAR [wiki](https://wiki.near.org/)!
|
---
id: access-keys
title: Access Keys
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
The RPC API enables you to retrieve information about an account's access keys.
---
## View access key {#view-access-key}
Returns information about a single access key for given account.
If `permission` of the key is `FunctionCall`, it will return more details such as the `allowance`, `receiver_id`, and `method_names`.
- method: `query`
- params:
- `request_type`: `view_access_key`
- [`finality`](/api/rpc/setup#using-finality-param) _OR_ [`block_id`](/api/rpc/setup#using-block_id-param)
- `account_id`: _`"example.testnet"`_
- `public_key`: _`"example.testnet's public key"`_
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "query",
"params": {
"request_type": "view_access_key",
"finality": "final",
"account_id": "client.chainlink.testnet",
"public_key": "ed25519:H9k5eiU4xXS3M4z8HzKJSLaZdqGdGwBG49o7orNC4eZW"
}
}
```
</TabItem>
<TabItem value="js" label="🌐 JavaScript" label="JavaScript">
```js
const response = await near.connection.provider.query({
request_type: "view_access_key",
finality: "final",
account_id: "client.chainlink.testnet",
public_key: "ed25519:H9k5eiU4xXS3M4z8HzKJSLaZdqGdGwBG49o7orNC4eZW",
});
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=query \
params:='{
"request_type": "view_access_key",
"finality": "final",
"account_id": "client.chainlink.testnet",
"public_key": "ed25519:H9k5eiU4xXS3M4z8HzKJSLaZdqGdGwBG49o7orNC4eZW"
}'
```
</TabItem>
</Tabs>
<details>
<summary>Example response: </summary>
<p>
```json
{
"jsonrpc": "2.0",
"result": {
"nonce": 85,
"permission": {
"FunctionCall": {
"allowance": "18501534631167209000000000",
"receiver_id": "client.chainlink.testnet",
"method_names": ["get_token_price"]
}
},
"block_height": 19884918,
"block_hash": "GGJQ8yjmo7aEoj8ZpAhGehnq9BSWFx4xswHYzDwwAP2n"
},
"id": "dontcare"
}
```
</p>
</details>
#### What Could Go Wrong?
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `view_access_key` request type:
<table className="custom-stripe">
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr>
<td rowspan="6">HANDLER_ERROR</td>
<td>UNKNOWN_BLOCK</td>
<td>The requested block has not been produced yet or it has been garbage-collected (cleaned up to save space on the RPC node)</td>
<td>
<ul>
<li>Check that the requested block is legit</li>
<li>If the block had been produced more than 5 epochs ago, try to send your request to <a href="https://near-nodes.io/intro/node-types#archival-node">an archival node</a></li>
</ul>
</td>
</tr>
<tr>
<td>INVALID_ACCOUNT</td>
<td>The requested <code>account_id</code> is invalid</td>
<td>
<ul>
<li>Provide a valid <code>account_id</code></li>
</ul>
</td>
</tr>
<tr>
<td>UNKNOWN_ACCOUNT</td>
<td>The requested <code>account_id</code> has not been found while viewing since the account has not been created or has been already deleted</td>
<td>
<ul>
<li>Check the <code>account_id</code></li>
<li>Specify a different block or retry if you request the latest state</li>
</ul>
</td>
</tr>
<tr>
<td>UNKNOWN_ACCESS_KEY</td>
<td>The requested <code>public_key</code> has not been found while viewing since the public key has not been created or has been already deleted</td>
<td>
<ul>
<li>Check the <code>public_key</code></li>
<li>Specify a different block or retry if you request the latest state</li>
</ul>
</td>
</tr>
<tr>
<td>UNAVAILABLE_SHARD</td>
<td>The node was unable to found the requested data because it does not track the shard where data is present</td>
<td>
<ul>
<li>Send a request to a different node which might track the shard</li>
</ul>
</td>
</tr>
<tr>
<td>NO_SYNCED_BLOCKS</td>
<td>The node is still syncing and the requested block is not in the database yet</td>
<td>
<ul>
<li>Wait until the node finish syncing</li>
<li>Send a request to a different node which is synced</li>
</ul>
</td>
</tr>
<tr className="stripe">
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
<tr>
<td>INTERNAL_ERROR</td>
<td>INTERNAL_ERROR</td>
<td>Something went wrong with the node itself or overloaded</td>
<td>
<ul>
<li>Try again later</li>
<li>Send a request to a different node</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
---
## View access key list {#view-access-key-list}
You can query <strong>all</strong> access keys for a given account.
- method: `query`
- params:
- `request_type`: `view_access_key_list`
- [`finality`](/api/rpc/setup#using-finality-param) _OR_ [`block_id`](/api/rpc/setup#using-block_id-param)
- `account_id`: _`"example.testnet"`_
Example:
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "query",
"params": {
"request_type": "view_access_key_list",
"finality": "final",
"account_id": "example.testnet"
}
}
```
</TabItem>
<TabItem value="js" label="🌐 JavaScript" label="JavaScript">
```js
const response = await near.connection.provider.query({
request_type: "view_access_key_list",
finality: "final",
account_id: "example.testnet",
});
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=query \
params:='{
"request_type": "view_access_key_list",
"finality": "final",
"account_id": "example.testnet"
}'
```
</TabItem>
</Tabs>
<details>
<summary>Example response: </summary>
<p>
```json
{
"jsonrpc": "2.0",
"result": {
"keys": [
{
"public_key": "ed25519:2j6qujbkPFuTstQLLTxKZUw63D5Wu3SG79Gop5JQrNJY",
"access_key": {
"nonce": 17,
"permission": {
"FunctionCall": {
"allowance": "9999203942481156415000",
"receiver_id": "place.meta",
"method_names": []
}
}
}
},
{
"public_key": "ed25519:46etzhzZHN4NSQ8JEQtbHCX7sT8WByS3vmSEb3fbmSgf",
"access_key": {
"nonce": 2,
"permission": {
"FunctionCall": {
"allowance": "9999930655034196535000",
"receiver_id": "dev-1596616186817-8588944",
"method_names": []
}
}
}
},
{
"public_key": "ed25519:4F9TwuSqWwvoyu7JVZDsupPhC7oYbYNsisBV2yQvyXFn",
"access_key": {
"nonce": 0,
"permission": "FullAccess"
}
},
{
"public_key": "ed25519:4bZqp6nm1btr92UfKbyADDzJ4oPK9JetHXqEYqbYZmkD",
"access_key": {
"nonce": 2,
"permission": "FullAccess"
}
},
{
"public_key": "ed25519:6ZPzX7hS37jiU9dRxbV1Waf8HSyKKFypJbrnZXzNhqjs",
"access_key": {
"nonce": 2,
"permission": {
"FunctionCall": {
"allowance": "9999922083697042955000",
"receiver_id": "example.testnet",
"method_names": []
}
}
}
},
{
"public_key": "ed25519:81RKfuo7mBbsaviTmBsq18t6Eq4YLnSi3ye2CBLcKFUX",
"access_key": {
"nonce": 8,
"permission": "FullAccess"
}
},
{
"public_key": "ed25519:B4W1oAYTcG8GxwKev8jQtsYWkGwGdqP24W7eZ6Fmpyzc",
"access_key": {
"nonce": 0,
"permission": {
"FunctionCall": {
"allowance": "10000000000000000000000",
"receiver_id": "dev-1594144238344",
"method_names": []
}
}
}
},
{
"public_key": "ed25519:BA3AZbACoEzAsxKeToFd36AVpPXFSNhSMW2R6UYeGRwM",
"access_key": {
"nonce": 0,
"permission": {
"FunctionCall": {
"allowance": "10000000000000000000000",
"receiver_id": "new-corgis",
"method_names": []
}
}
}
},
{
"public_key": "ed25519:BRyHUGAJjRKVTc9ZqXTTSJnFmSca8WLj8TuVe1wXK3LZ",
"access_key": {
"nonce": 17,
"permission": "FullAccess"
}
},
{
"public_key": "ed25519:DjytaZ1HZ5ZFmH3YeJeMCiC886K1XPYeGsbz2E1AZj2J",
"access_key": {
"nonce": 31,
"permission": "FullAccess"
}
},
{
"public_key": "ed25519:DqJn5UCq6vdNAvfhnbpdAeuui9a6Hv9DKYDxeRACPUDP",
"access_key": {
"nonce": 0,
"permission": "FullAccess"
}
},
{
"public_key": "ed25519:FFxG8x6cDDyiErFtRsdw4dBNtCmCtap4tMTjuq3umvSq",
"access_key": {
"nonce": 0,
"permission": "FullAccess"
}
}
],
"block_height": 17798231,
"block_hash": "Gm7YSdx22wPuciW1jTTeRGP9mFqmon69ErFQvgcFyEEB"
},
"id": "dontcare"
}
```
</p>
</details>
#### What Could Go Wrong?
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `view_access_key_list` request type:
<table className="custom-stripe">
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr>
<td rowspan="5">HANDLER_ERROR</td>
<td>UNKNOWN_BLOCK</td>
<td>The requested block has not been produced yet or it has been garbage-collected (cleaned up to save space on the RPC node)</td>
<td>
<ul>
<li>Check that the requested block is legit</li>
<li>If the block had been produced more than 5 epochs ago, try to send your request to <a href="https://near-nodes.io/intro/node-types#archival-node">an archival node</a></li>
</ul>
</td>
</tr>
<tr>
<td>INVALID_ACCOUNT</td>
<td>The requested <code>account_id</code> is invalid</td>
<td>
<ul>
<li>Provide a valid <code>account_id</code></li>
</ul>
</td>
</tr>
<tr>
<td>UNKNOWN_ACCOUNT</td>
<td>The requested <code>account_id</code> has not been found while viewing since the account has not been created or has been already deleted</td>
<td>
<ul>
<li>Check the <code>account_id</code></li>
<li>Specify a different block or retry if you request the latest state</li>
</ul>
</td>
</tr>
<tr>
<td>UNAVAILABLE_SHARD</td>
<td>The node was unable to find the requested data because it does not track the shard where data is present</td>
<td>
<ul>
<li>Send a request to a different node which might track the shard</li>
</ul>
</td>
</tr>
<tr>
<td>NO_SYNCED_BLOCKS</td>
<td>The node is still syncing and the requested block is not in the database yet</td>
<td>
<ul>
<li>Wait until the node finish syncing</li>
<li>Send a request to a different node which is synced</li>
</ul>
</td>
</tr>
<tr className="stripe">
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
<tr>
<td>INTERNAL_ERROR</td>
<td>INTERNAL_ERROR</td>
<td>Something went wrong with the node itself or overloaded</td>
<td>
<ul>
<li>Try again later</li>
<li>Send a request to a different node</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
---
## View access key changes (single) {#view-access-key-changes-single}
Returns individual access key changes in a specific block. You can query multiple keys by passing an array of objects containing the `account_id` and `public_key`.
- method: `EXPERIMENTAL_changes`
- params:
- `changes_type`: `single_access_key_changes`
- `keys`: `[{ account_id, public_key }]`
- [`finality`](/api/rpc/setup#using-finality-param) _OR_ [`block_id`](/api/rpc/setup#using-block_id-param)
Example:
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "EXPERIMENTAL_changes",
"params": {
"changes_type": "single_access_key_changes",
"keys": [
{
"account_id": "example-acct.testnet",
"public_key": "ed25519:25KEc7t7MQohAJ4EDThd2vkksKkwangnuJFzcoiXj9oM"
}
],
"finality": "final"
}
}
```
</TabItem>
<TabItem value="js" label="🌐 JavaScript" label="JavaScript">
```js
const response = await near.connection.provider.experimental_changes({
changes_type: "single_access_key_changes",
keys: [
{
account_id: "example-acct.testnet",
public_key: "ed25519:25KEc7t7MQohAJ4EDThd2vkksKkwangnuJFzcoiXj9oM",
},
],
finality: "final",
});
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=EXPERIMENTAL_changes \
params:='{
"changes_type": "single_access_key_changes",
"keys": [
{
"account_id": "example-acct.testnet",
"public_key": "ed25519:25KEc7t7MQohAJ4EDThd2vkksKkwangnuJFzcoiXj9oM"
}
],
"finality": "final"
}'
```
</TabItem>
</Tabs>
<details>
<summary>Example response: </summary>
<p>
```json
{
"jsonrpc": "2.0",
"result": {
"block_hash": "4kvqE1PsA6ic1LG7S5SqymSEhvjqGqumKjAxnVdNN3ZH",
"changes": [
{
"cause": {
"type": "transaction_processing",
"tx_hash": "HshPyqddLxsganFxHHeH9LtkGekXDCuAt6axVgJLboXV"
},
"type": "access_key_update",
"change": {
"account_id": "example-acct.testnet",
"public_key": "ed25519:25KEc7t7MQohAJ4EDThd2vkksKkwangnuJFzcoiXj9oM",
"access_key": {
"nonce": 1,
"permission": "FullAccess"
}
}
}
]
},
"id": "dontcare"
}
```
</p>
</details>
#### What Could Go Wrong?{#what-could-go-wrong-2}
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `EXPERIMENTAL_changes_in_block` method:
<table className="custom-stripe">
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr>
<td rowspan="2">HANDLER_ERROR</td>
<td>UNKNOWN_BLOCK</td>
<td>The requested block has not been produced yet or it has been garbage-collected (cleaned up to save space on the RPC node)</td>
<td>
<ul>
<li>Check that the requested block is legit</li>
<li>If the block had been produced more than 5 epochs ago, try to send your request to <a href="https://near-nodes.io/intro/node-types#archival-node">an archival node</a></li>
</ul>
</td>
</tr>
<tr>
<td>NOT_SYNCED_YET</td>
<td>The node is still syncing and the requested block is not in the database yet</td>
<td>
<ul>
<li>Wait until the node finish syncing</li>
<li>Send a request to a different node which is synced</li>
</ul>
</td>
</tr>
<tr className="stripe">
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
<tr>
<td>INTERNAL_ERROR</td>
<td>INTERNAL_ERROR</td>
<td>Something went wrong with the node itself or overloaded</td>
<td>
<ul>
<li>Try again later</li>
<li>Send a request to a different node</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
---
## View access key changes (all) {#view-access-key-changes-all}
Returns changes to <strong>all</strong> access keys of a specific block. Multiple accounts can be quereied by passing an array of `account_ids`.
- method: `EXPERIMENTAL_changes`
- params:
- `changes_type`: `all_access_key_changes`
- `account_ids`: `[ "example.testnet", "example2.testnet"]`
- [`finality`](/api/rpc/setup#using-finality-param) _OR_ [`block_id`](/api/rpc/setup#using-block_id-param)
Example:
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "EXPERIMENTAL_changes",
"params": {
"changes_type": "all_access_key_changes",
"account_ids": ["example-acct.testnet"],
"block_id": "4kvqE1PsA6ic1LG7S5SqymSEhvjqGqumKjAxnVdNN3ZH"
}
}
```
</TabItem>
<TabItem value="js" label="🌐 JavaScript" label="JavaScript">
```js
const response = await near.connection.provider.experimental_changes({
changes_type: "all_access_key_changes",
account_ids: "example-acct.testnet",
finality: "final",
});
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=EXPERIMENTAL_changes \
params:='{
"changes_type": "all_access_key_changes",
"account_ids": ["example-acct.testnet"],
"block_id": "4kvqE1PsA6ic1LG7S5SqymSEhvjqGqumKjAxnVdNN3ZH"
}'
```
</TabItem>
</Tabs>
<details>
<summary>Example response: </summary>
<p>
```json
{
"jsonrpc": "2.0",
"result": {
"block_hash": "4kvqE1PsA6ic1LG7S5SqymSEhvjqGqumKjAxnVdNN3ZH",
"changes": [
{
"cause": {
"type": "transaction_processing",
"tx_hash": "HshPyqddLxsganFxHHeH9LtkGekXDCuAt6axVgJLboXV"
},
"type": "access_key_update",
"change": {
"account_id": "example-acct.testnet",
"public_key": "ed25519:25KEc7t7MQohAJ4EDThd2vkksKkwangnuJFzcoiXj9oM",
"access_key": {
"nonce": 1,
"permission": "FullAccess"
}
}
},
{
"cause": {
"type": "receipt_processing",
"receipt_hash": "CetXstu7bdqyUyweRqpY9op5U1Kqzd8pq8T1kqfcgBv2"
},
"type": "access_key_update",
"change": {
"account_id": "example-acct.testnet",
"public_key": "ed25519:96pj2aVJH9njmAxakjvUMnNvdB3YUeSAMjbz9aRNU6XY",
"access_key": {
"nonce": 0,
"permission": "FullAccess"
}
}
}
]
},
"id": "dontcare"
}
```
</p>
</details>
#### What Could Go Wrong?{#what-could-go-wrong-3}
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `EXPERIMENTAL_changes` method:
<table className="custom-stripe">
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr>
<td rowspan="2">HANDLER_ERROR</td>
<td>UNKNOWN_BLOCK</td>
<td>The requested block has not been produced yet or it has been garbage-collected (cleaned up to save space on the RPC node)</td>
<td>
<ul>
<li>Check that the requested block is legit</li>
<li>If the block had been produced more than 5 epochs ago, try to send your request to <a href="https://near-nodes.io/intro/node-types#archival-node">an archival node</a></li>
</ul>
</td>
</tr>
<tr>
<td>NOT_SYNCED_YET</td>
<td>The node is still syncing and the requested block is not in the database yet</td>
<td>
<ul>
<li>Wait until the node finish syncing</li>
<li>Send a request to a different node which is synced</li>
</ul>
</td>
</tr>
<tr className="stripe">
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
<tr>
<td>INTERNAL_ERROR</td>
<td>INTERNAL_ERROR</td>
<td>Something went wrong with the node itself or overloaded</td>
<td>
<ul>
<li>Try again later</li>
<li>Send a request to a different node</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
---
|
Illia Polosukhin returns to NEAR Foundation Council to continue the evolution of NEAR ecosystem, Diogo Mónica, Founder of Anchorage, joins as Chairman
NEAR FOUNDATION
October 18, 2023
NEAR Foundation today announced that Illia Polosukhin, co-founder of the NEAR protocol, will be returning to the NEAR Foundation Council (NFC) with immediate effect as part of a rotation of the NFC on the Foundation’s fourth anniversary. As an NFC member, Illia will be better positioned to support the evolution of the NEAR ecosystem, which puts Web3 founders at the core. Key developments will be revealed at this year’s NEARCON in Lisbon, 7-10 November.
As part of this rotation, Diogo Mónica has been appointed Chair of the NFC. Diogo is the Founder and President of Anchorage Digital, the world’s most regulated and proven institutional custodian. As the new Chair, Diogo brings years of experience in corporate leadership and crypto technologies, and a track record of scaling innovative and diverse teams in the digital asset space. Richard Muirhead, Founder, Chairman and Managing Partner at Fabric, will remain as NFC member, a position he has held since its inception; Sheila Warren, CEO of the Crypto Council for Innovation, will remain as advisor to the NFC; and Chris Donovan, CEO of NEAR Foundation, remains as NFC member.
Mona El Isa, Marieke Flament & Jason Warner have elected to step down from their positions on the NFC.
Diogo Mónica, NFC Chairperson, remarked: “I am delighted to be joining the NEAR Foundation Council as Chair, joining Illia, Chris, Richard and Sheila. I have been following the impressive progress of the NEAR ecosystem for some time. Huge strides have been made over the last few years in building towards an Open Web. I am privileged to play my part in the next evolution of the NEAR ecosystem to continue on the Open Web journey, and excited to do my bit to bring new ideas and direction to support Founders building in the NEAR ecosystem.”
The next evolution of the NEAR ecosystem will be unveiled at NEARCON, NEAR’s yearly ecosystem flagship event in Lisbon, from 7-10 November. Tickets are on sale at nearcon.org for $99.
|
---
id: education-resources
title: Learning Resources
sidebar_label: Resources
sidebar_position: 3
---
# Learning Resources
---
### [](https://www.freecodecamp.org/news/near-curriculum/)<svg width="13.5" height="13.5" aria-hidden="true" viewBox="0 0 24 24" class="link__logo iconExternalLink_node_modules-@docusaurus-theme-classic-lib-next-theme-IconExternalLink-styles-module"><path fill="currentColor" d="M21 13v10h-21v-19h12v2h-10v15h17v-8h2zm3-12h-10.988l4.035 4-6.977 7.07 2.828 2.828 6.977-7.07 4.125 4.172v-11z"></path></svg>
A fun, casual, introduction to NEAR Protocol and its underlying tech. Learn about NEAR, how it works, and how to build on it.
### [](https://www.freecodecamp.org/news/near-curriculum/)<svg width="13.5" height="13.5" aria-hidden="true" viewBox="0 0 24 24" class="link__logo iconExternalLink_node_modules-@docusaurus-theme-classic-lib-next-theme-IconExternalLink-styles-module"><path fill="currentColor" d="M21 13v10h-21v-19h12v2h-10v15h17v-8h2zm3-12h-10.988l4.035 4-6.977 7.07 2.828 2.828 6.977-7.07 4.125 4.172v-11z"></path></svg>
A freeCodeCamp initiative rolling out courses on NEAR Protocol.
### [](https://learnnear.club/)<svg width="13.5" height="13.5" aria-hidden="true" viewBox="0 0 24 24" class="link__logo iconExternalLink_node_modules-@docusaurus-theme-classic-lib-next-theme-IconExternalLink-styles-module"><path fill="currentColor" d="M21 13v10h-21v-19h12v2h-10v15h17v-8h2zm3-12h-10.988l4.035 4-6.977 7.07 2.828 2.828 6.977-7.07 4.125 4.172v-11z"></path></svg>
All-inclusive hands-on onboarding platform to NEAR Protocol – connecting NEAR Ecosystem projects to pro-active community members via (L)Earn method.
Supported by NEAR Foundation.
LNC also has some pretty great invite-only offers to help NEAR Ecosystem projects to bring their products to thousands of NEAR users via the [LNC for Projects](https://learnnear.club/lnc-for-projects-publish/) initiative!
* Earn NEAR by learning about the tech and ecosystem
* While interacting on LNC, you will receive [nLearns](https://learnnear.club/what-are-nlearns/), LNC internal points.
* Both Creators and Learners get rewards in nLEARNs
### [](https://near.academy/)<svg width="13.5" height="13.5" aria-hidden="true" viewBox="0 0 24 24" class="link__logo iconExternalLink_node_modules-@docusaurus-theme-classic-lib-next-theme-IconExternalLink-styles-module"><path fill="currentColor" d="M21 13v10h-21v-19h12v2h-10v15h17v-8h2zm3-12h-10.988l4.035 4-6.977 7.07 2.828 2.828 6.977-7.07 4.125 4.172v-11z"></path></svg>
This resource is aimed at developers with experience in Web 2, who are new to Web 3 [NEAR Academy](https://near.academy).
It's an interactive course that teaches how to integrate blockchain technology with an application.
When you complete it, you receive a certificate of completion.
## Crypto-immersion Training Course
We are going to take you through a **Crypto-immersion Training Course** prepared by NEAR Foundation.
These resources are intended to support you as you onboard to Web3. Whether you are starting a business or joining a team, these courses will help you find your bearings in the crypto space. Please take your time and go through each of the 4 courses outlined below. They Include:
* [Web 3 Basics](#web-3-basics)
* Brief breakdown of common terminology and how blockchain works at a high level
* [Understanding Web3 Bootcamp](/support/learning/understanding-web3/history/1.1_history-of-the-internet)
* Take a deeper dive into Web3 in this 6 part lecture series covering the history of the internet all the way to the metaverse
* [An introduction Course for Non-Programers](#an-introduction-course-to-near-for-non-programmers)
* Very simple, high level explanation of Web3 starting with how a computer works to how they combine to create sophisticated networks like blockchains
* [Becoming Crypto Savvy](#becoming-crypto-savvy)
* Collection of activities to help you understand and reason about the components that make up Web3
---
### Web 3 Basics
An **introduction to Web3**, the blockchain and NEAR
What is Web3? What is blockchain? What is NEAR?
**Learning Outcomes**
A novice new to Web3 will be able to:
* Describe what a blockchain is in a nutshell
* Discuss the difference between Web1.0, 2.0, and 3.0
* Learn about what decentralization is and why it is fundamental to the future of the open web
[Learn](https://near.org/learn/)
---
### An introduction course to NEAR for Non-Programmers
An **Intro course** to NEAR for **non-programmers** and those new to Web3.
**Learning Outcomes**
A novice to Web3 will be able to:
* Confidently describe the idea of the open web
* Confidently explain broad blockchain concepts
* Identify core features of any smart contract (view function, change function, etc.) ❤️
* Discuss identity, governance, and ownership in Web3
[NEAR and Far from Any Coding](https://nearandfar.io/)
---
### Becoming Crypto Savvy! Immersion Training Course
Back when NEAR conducted certification courses for aspiring blockchain developers, this collection of resources and activities was the prework required before attending the course. Although, the certification program has been sunsetted, this valuable document will help you really get into the weeds of how smart contracts work.
**Learning Outcomes**
A technical person new to Web3 will be able to:
* Create an account on NEAR Protocol
* Reason about smart contracts
* Understand the history of Bitcoin and its implications on future economies
* Learn to see this new world we are now living in
Please complete as many of these core activities as possible before we meet. The more of this you finish, the clearer your picture of our time together will be. Of course, the learning experience will be more fun as well if you are better prepared.
Good luck and have fun!
[NEAR Certified Programs - Welcome to Web3 - HackMD](https://hackmd.io/@nearly-learning/ncd-day-0)
---
**Other Resources**
[NEAR BOS Tutorials](https://docs.near.org/discovery/tutorial/quickstart)
[Hello from NEAR Wiki | NEAR Wiki](https://wiki.near.org/)
[The NEAR White Paper](https://near.org/papers/the-official-near-white-paper/)
|
A Commitment to Communication
NEAR FOUNDATION
November 8, 2022
The NEAR Foundation has always held transparency as one of its core beliefs. Being open to the community, investors, builders and creators is one of the core tenets of being a Web3 project. But it’s become apparent the Foundation needs to do more.
The Foundation hears the frustration from the community, and it wants to be more pro-active in when and how it communicates.
Below is a high level overview of its new communication strategy. More specific details will follow.
Relaunching town halls
Townhalls have been a tool the Foundation has used to communicate its strategy and highlight what the ecosystem has been up to in the past. These will be brought back in an updated format monthly that will focus more on showcasing what’s happening internally at the Foundation, and providing opportunities for projects to play a bigger role in decision making through a Q&A format.
A commitment to regular AMAs
The leadership team will be hosting fortnightly regular AMAs for the community to ask questions around strategy and future direction. The Foundation will also host specific AMAs around key parts of the Foundation to allow the community to ask questions to specific members of the team.
These AMAs will initially be focused around:
Marketing & Events
Community
BD/Funding
Education
Legal
Always on signposting
The Foundation has created lots of resources to help the community navigate resources and where to go for help. However, the Foundation is aware these could be sign-posted better. As such, it will shift to an always-on approach to better highlight where people can find the information they need, or speak to someone from the Foundation.
This is just the beginning of this strategy and Foundation will publish more updates on this approach in the coming weeks.
|
<br />
<br />
<p align="center">
<img src="website/static/img/near_logo.svg" width="400">
</p>
<br />
<br />
## NEAR Protocol - scalable and usable blockchain
[](http://near.chat)
[](https://github.com/near/node-docs/actions/workflows/build.yml)
* ⚖️ NEAR Protocol is a new smart-contract platform that delivers scalability and usability.
* 🛠 Through sharding, it will linearly scale with the number of validation nodes on the network.
* 🗝 Leveraging WebAssembly (via Rust and AssemblyScript), more sane contract management, ephemeral accounts and many other advancements, NEAR
finally makes using a blockchain protocol easy for both developers and consumers.
## Quick start
Check out the following links
- Deployed, live node documentation: https://near-nodes.io
- Example applications: https://near.dev
- Community chat: https://near.chat
## Contributing
NEAR uses [Docusaurus](https://docusaurus.io) for documentation. Please refer to their documentation for details on major structural contributions to the documentation.
For simple content changes you have 2 options
- [Submit an issue](https://github.com/near/node-docs/issues)
- [Submit a pull request](https://github.com/near/node-docs/pulls) *(we prefer PRs of course)*
### The instant PR
This is the fastest way to submit content changes directly from the page where you notice a mistake.
1. Open any page in the docs on https://near-nodes.io
2. Click the `[ Edit ]` button at the top right hand side of _every_ content page
3. Make your edits to the document that opens in GitHub by clicking the ✎ (pencil) icon
4. Submit a PR with your changes and comments for context
### The typical PR
This is the standard fork-branch-commit workflow for submitting pull requests to open source repositories
1. Fork this repo to your own GitHub account (or just clone it directly if you are currently a member of NEAR)
2. Open your editor to the _top level repo folder_ to view the directory structure as seen below
3. Move into the `/website` folder where you will run the following commands:
- Make sure all the dependencies for the website are installed:
```sh
# Install dependencies
yarn
```
- Run the local docs development server
```sh
# Start the site
yarn start
```
_Expected Output_
```sh
# Website with live reload is started
Docusaurus server started on port 3000
```
The website for docs will open your browser locally to port `3000`
4. Make changes to the docs
5. Observe those changes reflected in the local docs
6. Submit a pull request with your changes
|
# Context API
Context API mostly provides read-only functions that access current information about the blockchain, the accounts
(that originally initiated the chain of cross-contract calls, the immediate contract that called the current one, the account of the current contract),
other important information like storage usage.
Many of the below functions are currently implemented through `data_read` which allows to read generic context data.
However, there is no reason to have `data_read` instead of the specific functions:
- `data_read` does not solve forward compatibility. If later we want to add another context function, e.g. `executed_operations`
we can just declare it as a new function, instead of encoding it as `DATA_TYPE_EXECUTED_OPERATIONS = 42` which is passed
as the first argument to `data_read`;
- `data_read` does not help with renaming. If later we decide to rename `signer_account_id` to `originator_id` then one could
argue that contracts that rely on `data_read` would not break, while contracts relying on `signer_account_id()` would. However
the name change often means the change of the semantics, which means the contracts using this function are no longer safe to
execute anyway.
However there is one reason to not have `data_read` -- it makes `API` more human-like which is a general direction Wasm APIs, like WASI are moving towards to.
---
```rust
current_account_id(register_id: u64)
```
Saves the account id of the current contract that we execute into the register.
###### Panics
- If the registers exceed the memory limit panics with `MemoryAccessViolation`;
---
```rust
signer_account_id(register_id: u64)
```
All contract calls are a result of some transaction that was signed by some account using
some access key and submitted into a memory pool (either through the wallet using RPC or by a node itself). This function returns the id of that account.
###### Normal operation
- Saves the bytes of the signer account id into the register.
###### Panics
- If the registers exceed the memory limit panics with `MemoryAccessViolation`;
- If called in a view function panics with `ProhibitedInView`.
###### Current bugs
- Currently we conflate `originator_id` and `sender_id` in our code base.
---
```rust
signer_account_pk(register_id: u64)
```
Saves the public key fo the access key that was used by the signer into the register.
In rare situations smart contract might want to know the exact access key that was used to send the original transaction,
e.g. to increase the allowance or manipulate with the public key.
###### Panics
- If the registers exceed the memory limit panics with `MemoryAccessViolation`;
- If called in a view function panics with `ProhibitedInView`.
###### Current bugs
- Not implemented.
---
```rust
predecessor_account_id(register_id: u64)
```
All contract calls are a result of a receipt, this receipt might be created by a transaction
that does function invocation on the contract or another contract as a result of cross-contract call.
###### Normal operation
- Saves the bytes of the predecessor account id into the register.
###### Panics
- If the registers exceed the memory limit panics with `MemoryAccessViolation`;
- If called in a view function panics with `ProhibitedInView`.
###### Current bugs
- Not implemented.
---
```rust
input(register_id: u64)
```
Reads input to the contract call into the register. Input is expected to be in JSON-format.
###### Normal operation
- If input is provided saves the bytes (potentially zero) of input into register.
- If input is not provided does not modify the register.
###### Returns
- If input was not provided returns `0`;
- If input was provided returns `1`; If input is zero bytes returns `1`, too.
###### Panics
- If the registers exceed the memory limit panics with `MemoryAccessViolation`;
###### Current bugs
- Implemented as part of `data_read`. However there is no reason to have one unified function, like `data_read` that can
be used to read all
---
```rust
block_index() -> u64
```
Returns the current block height from genesis.
---
```rust
block_timestamp() -> u64
```
Returns the current block timestamp (number of non-leap-nanoseconds since January 1, 1970 0:00:00 UTC).
---
```rust
epoch_height() -> u64
```
Returns the current epoch height from genesis.
---
```rust
storage_usage() -> u64
```
Returns the number of bytes used by the contract if it was saved to the trie as of the
invocation. This includes:
- The data written with `storage_*` functions during current and previous execution;
- The bytes needed to store the account protobuf and the access keys of the given account.
|
Los Angeles Football Club and Dropt Rewrite Fan Experience Playbook Utilizing NEAR Protocol
NEAR FOUNDATION
September 19, 2023
NEAR Foundation is excited to announce a new partnership between Los Angeles Football Club (LAFC) and Dropt — a fan engagement platform built on the NEAR Protocol — supporting the club’s vision to rewrite the playbook on the fan experience. The collaboration kicks off with LAFC Gold — a Loyalty Club designed to recognize Los Angeles FC’s most devoted fans with a wide range of unprecedented benefits.
Let’s explore what LAFC’s new fan engagement product, LAFC Gold, looks like and what it means for an open web.
Elevating the football fan experience with LAFC Gold benefits
In the sports worlds, fans love to be part of the action, and not just at the arena. Sports teams understand that fans like to feel that they are being seen, heard, and valued for their significant contributions to the organization and its athletes. And in the world of football (“soccer” to Americans), fanbases are truly global, making it important that sports franchises meet their fans wherever they are.
With its new Loyalty Club, LAFC is acknowledging their fans and bringing them new benefits and experiences, utilizing Dropt’s digital engagement platform and NEAR technology.
“LAFC Gold promises to make LAFC fans and supporters feel seen, heard, and valued wherever they are in the world,” said LAFC Co-President & CBO Larry Freedman. “Watching a match at BMO Stadium is one of the best sports experiences anyone can have. With LAFC Gold, we now have a way to connect, recognize, and reward anyone who supports the Black & Gold.”
LAFC Gold delivers on its promise with exclusive experiences
LAFC Gold kicked off with a ticket giveaway to the historic matchup between LAFC and Inter Miami, including the experience of holding the American Flag during the National Anthem before the match. And with the 2022 World Cup, 4-time Champions League, and 7-time Ballon d’Or winner Lionel Messi on the pitch for Inter Miami, this was a match not to be missed.
For the last three home matches of the season, including the momentous El Tráfico against LA Galaxy this past weekend, LAFC Gold has given away 4 premium tickets with pre-match field access to winning LAFC Gold Members. Unlocking your chance to win experiences and benefits like these for Real Salt Lake (10/1) and Minnesota United FC (10/4) is as easy as signing up and securing your LAFC Gold Membership.
With Dropt’s digital fan engagement platform, LAFC Gold will be accessible and easy for fans to join. The kickoff is just the first of what will be a dynamic program designed to create new ways for fans to engage with LAFC and its club partners. And by utilizing the NEAR Protocol, LAFC will ensure that the team will remain at the forefront of the open web’s cutting-edge technology by allowing LAFC to verify and track every transaction in a secure environment.
To experience LAFC Gold, visit gold.lafc.com and sign up for an Entry, Local, Global, or Youth Membership.
LAFC Season Ticket Members are automatically enrolled in the program.
|
---
title: Search and Discovery
description: Overview of the NEAR BOS search experience
sidebar_position: 3
---
# Universal Search
## Highlights
* A comprehensive search experience that is able to help users quickly find everything apps and components to people to connect with.
* It increases discoverability and usability in BOS by providing users with a Web2 style search experience.
### What it is:
Universal Search is a comprehensive search experience that allows users to navigate and explore the Blockchain Operating System (BOS) in a way that feels familiar and intuitive. With Universal Search, users can explore every part of the BOS to quickly discover what they need using the integrated search bar, filtering, and sorting panels. This enables users to easily discover people to connect with, apps to explore, relevant posts, reference docs, and components that can help them accelerate building.
### Why it matters:
One of Web3’s greatest benefits, decentralization, has also made it difficult to easily search and explore everything the space has to offer, creating information gaps for many users. It has been a challenge to find interesting projects to try, thought leaders to follow, people to connect with, and Web3 events to attend. Users often currently rely on the “Alphas” they know as a jumping off point for referrals, this is both inefficient, and can often lead to questions about the accuracy and quality of the information.
Universal Search removes the Web3 information gap, by providing a simple, intuitive way to explore the BOS. Rather than having to dig through “Alphas”, threads, and forums to try to find information, users now have one trusted place to turn. This provides an easier, more accessible exploration and navigation experience for anyone in Web3, as well as those interested in entering the space. It also benefits developers, entrepreneurs, and projects across the BOS because it brings their work to a larger audience by making it easier to discover..
### Who it’s for:
* Developers - Having a unified search function helps developers onboard to NEAR with easily accessible guides, tutorials and docs, explore components and templates to jump start development, dig through transactions and actions for full project visibility, and follow and connect with other open web builders.
* Users - Universal search increases Web3 accessibility for users by making it easy to discover and explore interesting apps and products, connect with like minded people, and find trusted information and answers to questions.
### How it works:
* Type ahead and trending search
* Users tap the search bar and immediately see a typeahead dropdown showing the categories of information they would be able to search along with trending searches on alpha.near.org.
* As users type their search query, they see instant results displaying in the typeahead so that they can directly navigate to their destination.
* Full page results filtering
* When results are not immediately available in typeahead, or users want to see all related results from their search, they land a full page result view with a mix of data types and options to drill down.
* The filtering panel allows users to set parameters for their search to get results that best fit their needs, or use the sort mechanism to identify the most relevant results based on criteria like the most recent posts, most used component, or most followed users.
* Tech Stack
* There is extensive data indexing from the on-chain data that is structured in the carefully designed schema to power the fastest search experience.
* Query tagging is used to ensure the relevancy of the results and provide related search queries that users might be interested to use and explore.
* The search algorithm is constantly tuned to provide the most relevant results for users in the top of the list of results returned.
### What’s coming next:
* Content in app search
* New Data Types including gigs, events, and groups
* Discovery and recommendation experience |
---
sidebar_position: 2
---
# Terminology
## Abstraction definitions
### Chain
A *chain* is a replication machinery, that provides for any type of state a way to replicate across the network and reach consensus on the state.
|
Soon… | NEAR Update: June 14, 2019
COMMUNITY
June 14, 2019
We’re extremely close to getting our new consensus, Nightshade, deployed to TestNet! Over the last two weeks we’ve been merging big blocks of it into master branches repos that will be affected. Bear with us during the transition, there will be breaking changes. You can now send money in the wallet, as well as see and manage authorized applications. In addition, you can deploy contracts in Rust to our TestNet. Co-founder Illia has been traveling through China for talks and demos with the Web3 Foundation.
COMMUNITY AND EVENTS
We want everybody to be able to take part in building the decentralized web with us. So we’re sponsoring a Twitter pitch competition for the next 6 days to give away a free ticket to DWeb Camp. Check out the tweet above for how to enter.
Illia is in China! He has been presenting alongside Polkadot, Chainsafe, Edgeware and others in Shenzhen, Hangzhou, Shanghai and Beijing about NEAR, usability and sharding.
Great time with Illia from Near Protocol. pic.twitter.com/UNv5mzDj1l
— Kaikai Yang (@KKYohlala) June 14, 2019
We’ve partnered with the Web3 Foundation to host regular Twitter chats on topics relevant to the crypto community. Our first chat was on usability; the second on blockchain gaming. Tweet at us (@NEARProtocol) if you’d like to suggest a topic!
Upcoming events:
Alex is giving a talk on usability and scalability at IBM’s Blockchain Developer Summit on June 21 at Galvanize, San Francisco. Tickets available here.
Max is giving a talk on using Rust to build smart contracts at RustLab in Florence, Italy on June 29. Tickets available here.
Jess, Peter and Vlad will be running a Crypto-Prototyping Series at DWeb Camp, south of San Francisco (July 18-21). It will be a multi-day immersive experience for the decentralized web community run by the Internet Archive. Tickets available here.
Alex will be talking at the Web3 Summit in Berlin (August 21-25). Tickets available here.
WRITING AND CONTENT
We’re happy to release the two videos from the blockchain gaming panel and the DeFi panel we recorded in New York. We’ve also got a new whiteboard session with Monica from Kadena, and a new series we’re launching called Fireside Chats. These are going to cover war stories from blockchain founders, starting with blockchain gaming. Lastly, we released another article on avalanche. Links below!
Videos
Future of DeFi — Panel | NY Blockchain Week
Blockchain Blockchain Gaming in 2019 — Panel | NY Blockchain Week
Fireside Chat with Devin Finzer | NY Blockchain Week
Whiteboard Series Ep 19 with Monica Quaintance from Kadena
Whiteboard Series Ep 20 with John Pacific from NuCypher
We Sponsored Nueva Hacks. Here’s the video.
Writing
Avalanche vs The new IOTA consensus algorithm, with a touch of Spacemesh
ENGINEERING HIGHLIGHTS
We’ve had 58 PRs across 9 repos and 10 authors in the last two weeks. Featured repos: nearcore, nearlib, near-shell, and near-wallet. Don’t forget, it’s all open source.
Overall, a lot of progress in the app layer and merged Nightshade into master.
Application Layer
Wallet
Authorized apps management
Various UI fixes
Display proper username in profile
Send money fixes
Account recovery fixes
Fix login redirect (when recovering account / setting up new)
Nearlib
Setup CI for Nightshade integration
Refactored to TypeScript with relevant updates for Nightshade and cleaner and extensible API.
Support adding keys to accounts
AssemblyScript/bindings
Merge with latest upstream
AssemblyScript JSON
Update to work with latest upstream
Use as-pect for tests
Studio
Wait properly for compiler loading
Basic end-to-end tests for studio workflow (running on CI)
Rust Smart Contracts
We now support smart contracts written in Rust
Near CLI
Command syntax improvements
Attach tokens to calls
Send tokens
View account
Blockchain
Added self-call for contract-based account.
Major progress on separating large logical blocks into chunks for the new Nightshade sharding design (commits 1 and 2). Still WIP.
Switched to u128 for balances / stakes across the board.
Implemented validator rotation and staking transaction
Restored all integration tests
HOW YOU CAN GET INVOLVED
Join us! If you want to work with one of the most talented teams in the world right now to solve incredibly hard problems, check out our careers page for openings. And tell your friends ?
Learn more about NEAR in The Beginner’s Guide to NEAR Protocol. Stay up to date with what we’re building by following us on Twitter for updates, joining the conversation on Discord and subscribing to our newsletter to receive updates right to your inbox.
Reminder: you can help out significantly by adding your thoughts in our 2-minute Developer Survey.
https://upscri.be/633436/ |
---
id: contract-size
title: "Reducing Contract Size"
---
import {Github} from "@site/src/components/codetabs"
# Reducing a contract's size
## Advice & examples
This page is made for developers familiar with lower-level concepts who wish to reduce their contract size significantly, perhaps at the expense of code readability.
Some common scenarios where this approach may be helpful:
- contracts intended to be tied to one's account management
- contracts deployed using a factory
- future advancements similar to the EVM on NEAR
There have been a few items that may add unwanted bytes to a contract's size when compiled. Some of these may be more easily swapped for other approaches while others require more internal knowledge about system calls.
## Small wins
### Using flags
When compiling a contract make sure to pass flag `-C link-arg=-s` to the rust compiler:
```bash
RUSTFLAGS='-C link-arg=-s' cargo build --target wasm32-unknown-unknown --release
```
Here is the parameters we use for the most examples in `Cargo.toml`:
```toml
[profile.release]
codegen-units = 1
opt-level = "s"
lto = true
debug = false
panic = "abort"
overflow-checks = true
```
You may want to experiment with using `opt-level = "z"` instead of `opt-level = "s"` to see if generates a smaller binary. See more details on this in [The Cargo Book Profiles section](https://doc.rust-lang.org/cargo/reference/profiles.html#opt-level). You may also reference this [Shrinking .wasm Size](https://rustwasm.github.io/book/reference/code-size.html#tell-llvm-to-optimize-for-size-instead-of-speed) resource.
### Removing `rlib` from the manifest
Ensure that your manifest (`Cargo.toml`) doesn't contain `rlib` unless it needs to. Some NEAR examples have included this:
:::caution Adds unnecessary bloat
```toml
[lib]
crate-type = ["cdylib", "rlib"]
```
:::
when it could be:
:::tip
```toml
[lib]
crate-type = ["cdylib"]
```
:::
3. When using the Rust SDK, you may override the default JSON serialization to use [Borsh](https://borsh.io) instead. [See this page](contract-interface/serialization-interface.md#overriding-serialization-protocol-default) for more information and an example.
4. When using assertions or guards, avoid using the standard `assert` macros like [`assert!`](https://doc.rust-lang.org/std/macro.assert.html), [`assert_eq!`](https://doc.rust-lang.org/std/macro.assert_eq.html), or [`assert_ne!`](https://doc.rust-lang.org/std/macro.assert_ne.html) as these may add bloat for information regarding the line number of the error. There are similar issues with `unwrap`, `expect`, and Rust's `panic!()` macro.
Example of a standard assertion:
:::caution Adds unnecessary bloat
```rust
assert_eq!(contract_owner, predecessor_account, "ERR_NOT_OWNER");
```
:::
when it could be:
:::tip
```rust
if contract_owner != predecessor_account {
env::panic(b"ERR_NOT_OWNER");
}
```
:::
Example of removing `expect`:
:::caution Adds unnecessary bloat
```rust
let owner_id = self.owner_by_id.get(&token_id).expect("Token not found");
```
:::
when it could be:
:::tip
```rust
fn expect_token_found<T>(option: Option<T>) -> T {
option.unwrap_or_else(|| env::panic_str("Token not found"))
}
let owner_id = expect_token_found(self.owner_by_id.get(&token_id));
```
:::
Example of changing standard `panic!()`:
:::caution Adds unnecessary bloat
```rust
panic!("ERR_MSG_HERE");
```
:::
when it could be:
:::tip
```rust
env::panic_str("ERR_MSG_HERE");
```
:::
## Lower-level approach
For a `no_std` approach to minimal contracts, observe the following examples:
- [Tiny contract](https://github.com/near/nearcore/tree/1e7c6613f65c23f87adf2c92e3d877f4ffe666ea/runtime/near-test-contracts/tiny-contract-rs)
- [NEAR ETH Gateway](https://github.com/ilblackdragon/near-eth-gateway/blob/master/proxy/src/lib.rs)
- [This YouTube video](https://youtu.be/Hy4VBSCqnsE) where Eugene demonstrates a fungible token in `no_std` mode. The code for this [example lives here](https://github.com/near/core-contracts/pull/88).
- [Examples using a project called `nesdie`](https://github.com/austinabell/nesdie/tree/main/examples).
- Note that Aurora has found success using [rjson](https://crates.io/crates/rjson) as a lightweight JSON serialization crate. It has a smaller footprint than [serde](https://crates.io/crates/serde) which is currently packaged with the Rust SDK. See [this example of rjson](https://github.com/aurora-is-near/aurora-engine/blob/65a1d11fcd16192cc1bda886c62005c603189a24/src/json.rs#L254) in an Aurora repository, although implementation details will have to be gleaned by the reader and won't be expanded upon here. [This nesdie example](https://github.com/austinabell/nesdie/blob/bb6beb77e32cd54077ac54bf028f262a9dfb6ad0/examples/multisig/src/utils/json/vector.rs#L26-L30) also uses the [miniserde crate](https://crates.io/crates/miniserde), which is another option to consider for folks who choose to avoid using the Rust SDK.
:::note Information on system calls
<details>
<summary>Expand to see what's available from <code>sys.rs</code></summary>
<Github language="rust" start="" end="" url="https://github.com/near/near-sdk-rs/blob/master/near-sdk/src/environment/sys.rs" />
</details>
:::
|
NEAR Foundation Announces Policy Principles
NEAR FOUNDATION
April 28, 2023
It’s no surprise that the Web3 industry presents many unique challenges for lawmakers and regulators globally. Decentralised, permissionless technology is, in many ways, at odds with much of current law and regulation that assumes centralised, intermediated and gatekept systems. Adding to this inherent complexity, Web3 technology is borderless by nature, spanning countless jurisdictions and legal/regulatory frameworks.
We believe that fair, proportionate and clear regulation can benefit our industry and is necessary if we want to see genuine global growth and adoption of Web3 technology. We also believe that the policy outcomes advanced by most regulators globally (protecting investors, maintaining market integrity and promoting financial stability) are reasonable and capable of being achieved in the Web3 context with the right approach (i.e. regulatory equivalence is possible).
Indeed, many of Web3’s core features (including decentralisation, disintermediation, transparency and immutability) make it uniquely suited to effectively achieving these policy outcomes. But, to achieve these outcomes, regulation must be fair and proportionate. If regulation isn’t fair and proportionate — if it doesn’t consider the unique features and associated challenges inherent to Web3 technology — then it can stifle innovation and undermine the very policy goals lawmakers and regulators are trying to advance1.
CURRENT STATE OF PLAY
Currently, there is no common or consistent approach to Web3 regulation. Laws and regulations that do exist vary widely between jurisdictions. In some jurisdictions, including Switzerland, the European Union (EU), the United Kingdom (UK), Japan, Singapore and Hong Kong, we are seeing progress and, critically, a recognition that, at least in some respects, our industry does require a different approach. In the EU, for example, the Markets in Crypto-Assets Regulation (MiCA) (the text of which was agreed upon late last year and is anticipated to come into force in late 2024) aims to create a harmonised, comprehensive legal framework across all EU member states for the regulation of crypto assets and related activities2 (although other policy developments are troubling3). Switzerland was one of the first jurisdictions to implement an effective regulatory framework for digital assets back in 20184 and has since been home to a vibrant Web3 ecosystem. The UK has also signaled its intent to embrace our industry, recently launching a significant consultation to seek views on how the UK could best regulate the Web3 industry5, which followed an excellent Law Commission of England and Wales (Law Commission) paper on personal property law and digital assets (as well as a consultation on decentralised autonomous organisations (DAOs) which recently closed6).
However, in other jurisdictions, most notably the US, there has been an increasing, and in many cases, overt hostility towards our industry. Amongst other things, a recent White House report on crypto is scathing7, a Treasury Department analysis of decentralised finance (DeFi) (somewhat more balanced than the White House report) contains some fundamental technical and policy misunderstandings8, banking access for Web3 projects has been compromised in a seemingly coordinated and deliberate manner9, prominent lawmakers talk of creating an ‘anti-crypto army,’10 and aggressive legislation proposals are receiving increased support. Regulators continue to rely on outdated laws and regulations that aren’t fit for purpose without providing any clarity or guidance that the industry desperately needs, let alone a viable route to registration11. Amidst this complex and unclear regulatory background, regulators and the industry alike struggle with definitive asset classification and the industry faces a wave of enforcement actions12.
This approach is unquestionably damaging the Web3 industry in the US (and beyond), driving many projects out of the jurisdiction. It is not a fair or proportionate regulatory approach, it is regulation by enforcement.
WHERE DOES THIS LEAVE THE INDUSTRY?
There is much work to do, particularly in the US. As an industry, we need to recognise that these regulatory challenges and the apparent growing hostility can potentially harm us all. However, what is unfolding now across our industry in the US could become a seminal and net positive moment if we can unite and mobilise effectively.
The NEAR Foundation’s primary focus is to support the ongoing growth and development of the NEAR protocol and its associated ecosystem, but it also has a more general mandate to accelerate the adoption of open technologies at a global scale. The Foundation, therefore, has a responsibility to advocate for reasonable, proportionate and effective approaches to regulating our industry.
Part I of this series sets out the NEAR Foundation’s core policy priorities for the next 12 months and beyond to increase awareness of the key issues, and to show what guides the NEAR Foundation as we advocate on behalf of the NEAR ecosystem and the wider industry. These policy priorities are: (1) protect developers and open source software; (2) protect privacy; (3) promote autonomy; (4) protect validators; (5) promote clarity, collaboration and good faith engagement.
In Part II of this series, to follow shortly, we set out policy initiatives to help drive these priorities forwards.
NEAR FOUNDATION POLICY PRIORITIES
PROTECT DEVELOPERS AND OPEN SOURCE SOFTWARE
Developers are the lifeblood of our industry, responsible for creating, maintaining, and innovating the infrastructure on which Web3 operates. To foster innovation and growth, it is essential to safeguard developers from onerous regulation and ensure that publishing open source code remains free and open. Developers should be able to explore, experiment, and create without unnecessary constraints, burdensome obligations or fear of liability (including, for example, designation as fiduciaries13 or ‘Financial Institutions’14).
Open source code is a powerful tool that enables transparency, collaboration, and rapid innovation, allowing developers to share, modify, and build upon existing work while enhancing the security of the code. By defending the right to publish open source code, we also support the principles of freedom of expression and the free flow of information. This not only benefits the Web3 community but also fosters a culture of open collaboration across the entire technology industry and encourages its continued development.
PROTECT PRIVACY
Privacy is a fundamental human right and a cornerstone of the Web3 vision to create a more secure, user-centric and user-empowered web. However, as a neutral and highly transparent technology, blockchain (and other Web3 technology) is equally capable of being misused for warrantless mass surveillance and censorship if appropriate privacy safeguards aren’t in place. We are already seeing various attempts to erode privacy in Web3 that could have far-reaching consequences well beyond our industry15.
Web3 technology has demonstrated that maintaining privacy can be compatible with other important policy outcomes including, for example, the prevention of crime. Zero-knowledge proof (and other) innovations are already capable of implementing (for example) KYC/AML and sanctions checks without exposing sensitive personal data16.
Privacy should be staunchly defended to protect individuals from unwarranted surveillance, invasive data collection, censorship and malicious actors. Policymakers should support these outcomes when it comes to Web3 technology, as they have done in Web2 with data protection legislation such as GDPR and DPA. By championing privacy, we want to ensure that Web3 technology continues to respect users and empower them to own and control their data.
PROMOTE AUTONOMY
Ensuring individuals can use self-hosted products and services free of heavy restrictions or obligations is critical to user empowerment. Through self-hosting, users can fully control their assets and data, enhance their privacy, and reduce censorship risks and reliance on centralised intermediaries and service providers (and it is often within such centralised intermediaries that risk is most highly concentrated17). Self-hosting is also a foundational component of secure, resilient, diverse and competitive ecosystems since it minimises centralised points of failure and dangerous concentrations of power18 and supports decentralised infrastructure and governance systems19. But, we are already seeing proposals that would harm autonomy by significantly constraining the development and use of self-hosted products and services20.
Users should be able to seamlessly embrace self-hosting as a viable and attractive alternative to centralised service offerings and also safely participate in decentralised autonomous governance systems (including DAOs) without fear of liability or being subjected to burdensome obligations. Many in the industry, including those working on NEAR protocol’s transition to the Blockchain Operating System21, are pioneering technological innovations to make this possible. Policymakers should also enable these outcomes.
PROTECT VALIDATORS
Validators are the backbone of decentralised networks, ensuring those networks can function effectively by validating transactions, maintaining transaction accuracy and ensuring adherence to consensus rules. In performing this essential function, validators should not be subject to burdensome obligations or significant liability risks22.Accordingly, a balance should be struck between the need for regulatory clarity, network security and ease of validator participation.
A good starting point could be the creation of a light-touch, standardised public disclosure regime whereby validators would be required to disclose relevant information about their operations. This relevant information could include (amongst other things) relevant digital asset holdings, ownership of/the extent of their node operations, fee structure, affiliations with other significant ecosystem participants, hardware and software infrastructure, security measures, and past performance records. De minimis thresholds could be implemented so that the disclosure requirements would apply only to those validators operating at scale and in connection with significant networks. This approach could also provide much-needed clarity by confirming the nature and extent of participating validator obligations and liability in the context of network failures, security breaches or other adverse events, providing an incentive for participation in the regime.
This type of disclosure-based approach would reduce information asymmetry, and therefore promote investor protection and help to maintain market integrity. It also has other advantages, including: (1) focusing on the types of activities that are being undertaken rather than the nature or classification of the underlying asset (the latter approach can create enormous complexity, as evidenced by the current situation in the US); and (b) being relatively flexible and so capable of application to other critical network participants including, for example, core development teams who are building/maintaining certain types of Web3 projects/infrastructure23.
By establishing clear guidelines and legal safeguards for validators (and other network participants), policymakers can provide a conducive environment for validators to operate without fear of significant legal consequences. This will promote the resilience, security and growth of decentralized networks and help to preserve critical benefits of Web3 – decentralisation and disintermediation.
PROMOTE CLARITY, COLLABORATION AND GOOD FAITH ENGAGEMENT
The industry desperately needs clarity from lawmakers and regulators. Effective policy debate requires willingness from both policymakers, and the industry to discuss issues in good faith24, as well as a recognition that Web3 presents a unique and unprecedented policy challenge and therefore an openness to exploring new approaches.
We should also recognise that as developers, users, and other proponents of Web3 technology, we are each representatives of and advocates for our industry. Our conduct matters, and we should all strive to act with integrity and set a good example to show policymakers what our industry is capable of, and the unique value it can generate25. To do this effectively, collaboration across the industry is essential.
CLOSING THOUGHTS
Productive dialogue between the industry and regulators will hopefully lead towards further legal and regulatory clarity in Web3, which will have a positive effect on: (1) developers, by offering them a framework in which to operate, aware of their obligations and protected from the unreasonable application of unsuitable laws and regulations; and (2) users, by providing a safe environment in which to try new products and services and explore a new technological frontier where autonomy, transparency and privacy are paramount.
We believe that the light-touch disclosure based approaches (like the high-level proposal referenced above), as well as safe harbour implementations26 (which provide projects with viable, conditioned routes to registration/compliance – and which could be integrated into a wider disclosure based regime), sandboxes (to explore specific activities like digital asset issuance, updated AML/KYC processes etc.) and model law proposals27 (amongst other approaches), show significant promise and warrant further work and discussion28.
We will explore some of these potential routes forwards in more detail in Part II of this series.
In the meantime, we believe that regulatory clarity and consensus are essential for the meaningful global adoption of Web3 technology. Policymakers’ approach to Web3 will continue to evolve, and on-going education efforts and good faith discussions will be key to moving the conversation in a positive direction. We welcome the opportunity to engage with other industry participants, as well as lawmakers and regulators globally, to develop a fair and proportionate approach in support of these policy priorities.
Disclaimer: Nothing in this article/post should be construed as legal, tax or investment advice. This post might not reflect all current updates to applicable laws, regulations or guidance. The authors disclaim any obligation to update this post and reserve the right to make any changes to this post without notice. In all cases, persons should conduct their own investigation and analysis of the information in this post. Where this post contains links to other sites and resources provided by third parties, these links are provided for information only and should not be interpreted as approval by us of those linked websites (or any information obtained from them) that the authors of this post do not control.
Footnotes:
1. See, e.g., Running on Empty: A Proposal to Fill the Gap Between Regulation and Decentralization, Hester M. Peirce, speech on February 6, 2020 (available here), where Peirce discusses the current challenges in the US with the lack of legal clarity around decentralization and the outdated application of the Howey Test to crypto assets. See also, e.g., The SEC, Digital Assets and Game Theory, Yuliya Guseva, 46 J. Corp.. L. 629-679 (2021)(available here), which suggests that the SEC’s regulation via enforcement actions approach has broken the ‘fabric of cooperation’ between industry participants and the SEC and is therefore leading to bad outcomes for all market participants. See further testimony before the US House Financial Services Subcommittee on Digital Assets, Financial Technology, and Inclusion, hearing entitled Coincidence or Coordinated? The Administrator’s Attack on the Digital Asset Ecosystem, Dr. Tonya M. Evans, March 9, 2023 (available here), where Evans emphasizes the difficulty in innovating and building in Web3 given the present uncertain regulatory climate in the US.
2. MiCA will primarily regulate centralised intermediaries/crypto-asset service providers in a relatively proportionate manner, enabling so-called ‘passporting’ across all member states and largely carving out decentralised initiatives, demonstrating a clear recognition by EU lawmakers and regulators of the current and future importance of our industry. MiCA isn’t perfect – the provisions governing stablecoins are unwieldy and challenging, the bloc’s intended approach to decentralised initiatives remains largely unknown and some other legislative developments are troubling (see footnote 3) – however, it represents a meaningful launching-off point for engagement and future iteration, and will provide much-needed clarity and consistency across the world’s largest single market.
3. See, e.g., Proposal for a Regulation on Information Accompanying transfers of funds and Certain Crypto-Assets, Piotr Bąkowski, European Parliamentary Research Service, 20 July 2021 (available here), which proposes to expand travel rule obligations to all crypto-asset service providers (CASPS) and require them to collect identifying data in respect of all transactions with no minimum transaction threshold (while the TradFi equivalent rule has a €1k minimum transaction threshold). See also, e.g., Proposal for a Regulation of the European Parliament and of the Council on harmonised rules on fair access to and use of data (Data Act), European Commission, 23 February 2022 (available here), which proposes mandating that certain smart contracts be ‘reversible’ and/or contain a so-called ‘kill switch’ in the ‘Internet of Things’ (IOT) context. See also, e.g., “Decentralised” or “disintermediated” finance: what regulatory response?, April 2023, the Autorité de Contrôle Prudentiel et de Résolution (ACPR) (available here), which proposes, in effect, to ‘re-intermediate’ DeFi (amongst other things) by requiring DeFi projects to incorporate in the EU, and to classify DeFi front-ends as regulated intermediaries with data collection and monitoring obligations.
4. The Swiss Financial Market Supervisory Authority (FINMA) published ‘ICO Guidelines’ in February 2018 (available here), which set out a relatively straightforward digital asset classification framework with the aim of providing clarity to market participants.
5. The consultation indicates an approach inspired partly by MiCA — i.e. regulate centralised intermediaries based on their activities, but recognise that decentralised initiatives will require a different approach — and asks the industry to provide views and feedback to inform the UK Government’s approach. This degree of engagement with industry is extremely encouraging. The NEAR Foundation will be submitting a consultation response and we would encourage all industry stakeholders to do the same. The consultation paper is available here.
6. The Law Commission’s consultation paper dealing with law reform proposals in the context of digital assets and personal property rights is available here, and the Law Commission’s consultation paper on DAOs can be found here.
7. The Annual Report of the Council of Economic Advisors, 20 March 2023 (available here), p. 238. (“[C]rypto assets to date do not appear to offer investments with any fundamental value, nor do they act as an effective alternative to fiat money, improve financial inclusion, or make payments more efficient; instead, their innovation has been mostly about creating artificial scarcity in order to support crypto assets’ prices—and many of them have no fundamental value.”)
8. See, e.g., Coin Centre’s excellent commentary on the Treasury DeFi risk assessment. Peter Van Valkenburgh, Treasury’s new DeFi risk assessment relies on ill-fitting frameworks and makes potentially unconstitutional recommendations, 7 April 2023 (available here).
9. See, e.g., Operation Choke Point 2.0: The Federal Bank Regulators Come for Crypto, Cooper & Kirk LLP (available here).
10. See, e.g., Elizabeth Warren is building an anti-crypto army. Some conservatives are on board, Zachary Warmbrodt, Politico, 14 February 2023 (available here).
11. See, e.g., Due to SEC Inaction, Registration is Not a Viable Path for Crypto Projects, Rodrigo Seira, Justin Slaughter, Katie Biber, Paradigm (available here), which notes that despite SEC Chair Genlser repeatedly telling projects to ‘come in and register’, current US securities laws are incompatible with various features of Web3, making registration a practical impossibility for Web3 projects.
12. Only this week, the SEC commenced an enforcement action against Bittrex, alleging, among other things that the Bittrex Platform “merged three functions that are typically separated in traditional securities markets—those of broker-dealers, exchanges, and clearing agencies—despite the fact that Bittrex has never registered with the SEC as a broker-dealer, national securities exchange, or clearing agency,” and arguing that five tokens, including Algorand and DASH, are securities. Securities and Exchange Commission v Bittrex Inc., (WD Wash) (Complaint), No 2:23 cv 00580. See also e.g., SEC Cryptocurrency Enforcement Update, Cornerstone Research 2022 (available here), according to which, in 2022 alone the SEC brought 30 crypto-related enforcement actions against Web3 projects. In spite of the numerous enforcement actions, the SEC has still not provided any material guidance to the industry to foster regulatory compliance. For a longer list of SEC crypto asset enforcement actions, please refer to the SEC’s summary available here.
13. See, e.g. Tulip Trading Ltd v Van der Laan and Ors [2023] EWCA Civ 83, a case currently in the High Court of England & Wales (following the successful appeal of a High Court order for summary judgment which was overturned by the Court of Appeal) that considers whether certain core developers of various Bitcoin networks owe network users either a fiduciary duty and/or a tortious duty of care, and therefore is one of the most important cases to date in the context of developer duties/liability.
14. See e.g., 31 U.S.C. § 5312(a)(2) (defining a financial institution as an entity encompassing various organizations and businesses, including but not limited to banks, credit unions, investment companies, broker-dealers, insurance companies, currency exchanges, and money transmitters, or deemed to engage in similar activities). Being classified as a ‘Financial Institution’ from a US law perspective subjects a person to (amongst other things) various onerous information collection and reporting duties, including a duty to: (1) identify and record personal information of any person who uses the relevant software/service; (2) develop anti-money laundering (AML) programmes that can block persons from using the relevant software/service where there are suspicions of illegal activity; and (3) file reports about users of the relevant software/service to the government.
15. See, e.g., the Digital Asset Anti-Money Laundering Act proposed in December last year in the US by Senators Warren and Marshall (the “Warren/Marshall Act”), which would significantly erode the privacy of anyone using or supporting public blockchain infrastructure; See also, e.g., Coin Centre’s post here for further analysis. See further, e.g., the US Infrastructure Bill which contains two particularly problematic revisions to the US Tax Code: (1) widening the definition of ‘broker’ so that it captures non-custodial service providers and transfers involving self-hosted wallets; and (2) an adjustment to include digital assets within provision §6050I, with the result that businesses would need to collect information about counterparties whenever they receive digital assets worth more than $10,000 in value. See also footnote 3 re ACPR’s proposals to treat DeFi front-ends as intermediaries which would require information collection and reporting with respect to users.
16. Zero-Knowledge (ZK) proofs are a cryptographic technique that enable one party to prove to another party that they have knowledge of a certain piece of information, without revealing the underlying information itself. ZK proofs can enhance privacy and efficiency in KYC and AML compliance, as they can enable individuals to prove that they have already completed KYC with a trusted provider without disclosing their personal data to other parties. ZK proofs can also allow individuals to prove that they are not on any sanctions or watch lists without revealing their identity or location. ZK proofs can reduce the risk of identity theft, data breaches, and fraud, as well as lower the operational costs and complexity of KYC and AML processes. See, e.g., zkKYC – A solution concept for KYC without knowing your customer, leveraging self-sovereign identity and zero-knowledge proofs, Pieter Pauels, Smart Contract Research Forum, July 2021 (available here).
17. For example, both FTX and Alameda were (extremely) centralised digital asset intermediaries, performing functions and offering services to customers in a bundled manner, and it is critical to recognise that: (1) the market contagion following the collapse of FTX/Alameda was significantly amplified precisely because of the centralised, opaque nature of the institutions (as well as alleged criminal behaviour); and (2) the contagion across decentralised ecosystems, which are open, transparent, and disintermediated by design, was notably less severe precisely because of these unique Web3 features. In relation to (2) see, e.g., Report of the Cotnell SC Johnson College of Business, 2022 Cornell Roundtable Forum on Digital Assets, (available here).
18. From a policy perspective, a key element of promoting autonomy involves the prevention of concentrations of power amongst a small number of centralised service providers/market actors that bundle various services together, something that has been a feature of the industry to date. See, e.g., Policy Paper Series (Part 1): Reframing How We Look at a Crypto Legislative Solution, Delphi Digital & LeXPunK, 17 March 2023 (available here)), which proposes a regulatory framework for public permissionless blockchain networks focussing on the regulation of systemically important vertically-integrated centralised actors.
19. The NEAR Digital Collective (NDC) is a community grassroots initiative in the NEAR ecosystem that is working to support the ongoing development of sustainable governance infrastructure and the ecosystem’s continuing decentralisation. The NEAR Foundation supported the NDC’s recent launch of a decentralised treasury that represents an important step in the NEAR ecosystem’s ongoing decentralisation. See, e.g., NEAR Digital Collective Legal Framework, 21 March 2023 (available here).
20. The Warren/Marshall Act would classify all providers of self-hosted wallets (and effectively anyone else who maintains public blockchain infrastructure) as ‘Financial Institutions’, subjecting them to a host of onerous obligations that are in practice impossible to comply with, and would significantly constrain the development and use of self-hosted products and services. See footnote 14 for more detail on the onerous obligations of ‘Financial Institutions’ from a US perspective. See also footnote 3 re ACPR’s proposals to treat DeFi font-ends as intermediaries which would require information collection and reporting with respect to users.
21. The Blockchain Operating System (BOS) serves as a single platform that developers can build into and users can interact on, including by browsing and discovering Web3 products such as crypto exchanges, non-fungible token (NFT) galleries and social networks. The BOS will be compatible with all blockchains (currently supporting NEAR protocol and Ethereum Virtual Machine chains), with NEAR protocol acting as the common entry point. The BOS offers a decentralized and composable and front end framework for building, launching, and using dApps, while leveraging common user experience frameworks such as profiles, notifications, and searching.For more information on the BOS, see, e.g., Near Protocol Announces the Blockchain Operating System, NEAR Foundation, 2 March 2023 (available here); Near Protocol Starts ‘Blockchain Operating System’ to Focus on User Experience, Lyllah Ledesma, Coindesk, 2 March 2023 (available here).
22. For example, Rule 3b-16(a)(2), Securities Exchange Act of 1934, 15 U.S.C. §§ 78a-78qq (2012). defines an exchange as an organization that (1) brings together the orders for securities of multiple buyers and sellers; and (2) uses established, non-discretionary methods under which such orders interact with each other, and the buyers and sellers entering such orders agree to the terms of the trade. The SEC’s proposed amendment to Rule 3b-16(a)(2) replaces the phrase “uses established, non-discretionary methods,” with “makes available established, non-discretionary methods,” which could inadvertently capture “developers working with all manners or protocols, front end systems, and smart contracts,” along with persons who “operate…’validators’ on the underlying blockchain where the AMM is stored”. See Reg-X-Proposal-An-Expempt-Offering-Framework-for-Token-Issuances, LeXpunK-Army, 7 March 2023 (available here).
23. The “Token Safe Harbor Proposal 2.0” framework proposed by SEC Commissioner Hester Peirce includes measures applying to validators, including “information, detailing…the process for validating transactions.” See Token Safe Harbor Proposal 2.0, Commissioner, Hester M. Peirce, 13 April 2021 (available here); See also, e.g., Safe Harbor X, LeXpunK-Army, 7 March 2023 (available here) (proposing a rule that would provide an exemption for the distribution of autonomous cryptotokens to users and builders of autonomous software systems, based on the Token Safe Harbor Proposal 2.0, but with some modifications and additions, such as requiring semi-annual updates, an exit report, guidance on decentralization criteria, and clarifying the definition and scope of autonomous cryptotokens and autonomous cryptosystems). These safe harbor proposals would operate to effectively provide projects with conditioned routes to compliance, based on each project meeting various requirements (including disclosures, smart contract audits, and degree of decentralisation).
24. In any principles-based regulatory system, regulators are generally afforded wide discretion to interpret and enforce regulations in line with desired policy outcomes. Where that discretion is misused or otherwise not exercised in good faith, those systems break down with the result that innovation is stifled and policy goals are undermined.
25. See, e.g., Community Policy Initiative No. 1, Polygon Labs, 11 April 2023 (available here).
26. See footnote 23 for more information on potential safe harbor implementations in the US.
27. Model law proposals are draft laws or regulations that are proposed by regulators or other authorities to address specific issues or challenges in a given domain. Model law consultations and proposals can serve as guidance or inspiration for other jurisdictions that are considering similar reforms or initiatives. See, e.g., Model Law For Decentralized Autonomous Organizations, Constance Choi, Primavera De Filippi, Rick Dudley, Silke Not Elrifia, Coalition of Automated Legal Applications, 2021 (available here); Proposal for a Regulation on Markets in Crypto-assets, European Commission, September 2020 (available here) (proposing a regulation that would establish a comprehensive framework for crypto-assets in the European Union, which eventually led to MiCA); Future financial services regulatory regime for cryptoassets: Consultation and call for evidence, UK Treasury, February 2023 (available here) (proposing a new regulatory regime for cryptoassets in the UK).
28. See, e.g.,Brief of LexpunK as Amicus Curiae Regarding Plainitff’s Motion for Alternative Service, LeXpunK, CFTC v. Ooki DAO et al., No. 3:22-cv-05416-WHO (N.D. Cal. Oct. 17, 2022) (available here) (challenging the CFTC’s motion for alternative service against token holders of Ooki DAO, a decentralized crypto platform, on the basis that DAOs are not legal entities or persons, but rather collections of smart contracts that run autonomously on a blockchain without any central authority or control). See also, e.g., Brief of Coinbase Global Inc. as Amicus Curiae in Support of Defendants’ Motion to Dismiss, Coinbase Global Inc., SEC v. Ripple Labs Inc. et al., No. 1:20-cv-10832-AT-SN (S.D.N.Y. Oct. 31, 2022) (available here) (arguing that the SEC failed to provide fair notice to market participants that XRP was considered a security); See also, e.g., Brief of Blockchain Association as Amicus Curiae in Support of Defendants’ Motion to Dismiss, Blockchain Association, SEC v. Wahi et al., No. 2:22-cv-02101-RSL (W.D. Wash. Feb. 13, 2023) (available here) (expressing support for the Defendants’ argument for dismissal and claiming that the SEC exceeded its authority by declaring certain tokens to be securities without prior findings and attempting to punish absent third parties who had no meaningful opportunity to defend themselves); See also, e.g.,Brief of Blockchain Association and DeFi Education Fund as Amici Curiae in Support of Plaintiffs’ Motion for Partial Summary Judgment, Blockchain Association & DeFi Education Fund, Van Loon v. Dep’t of the Treasury, No. 1:23-cv-00312-RP (W.D. Tex. April 12, 2023) (available here) (challenging OFAC’s sanctions on Tornado Cash, a privacy-protecting software protocol on Ethereum, as being based on a misunderstanding of blockchain technology and violating the Administrative Procedure Act). |
Nightshade | NEAR Update July 1, 2019
COMMUNITY
July 1, 2019
We deployed a test version of Nightshade, our new consensus model, and have been working to integrate it into the rest of our codebase.
This all happened while Illia was behind the Great Firewall, managing to both contribute code and give presentations to Chinese audiences on NEAR in collaboration with the Web3 foundation. On the ecosystem side, we have been heads down preparing some future programs to be announced soon.
We announced the winner of our DWeb Camp ticket giveaway, who won with an idea for a decentralized VPN (Well done Grace!).
COMMUNITY AND EVENTS
We are gearing up for another hackathon, and some other (secret!) programs for those who want to get more involved with NEAR, so stay tuned for updates in the coming weeks.
Alex gave a talk on usability and scalability at IBM’s Blockchain Developer Summit [https://blockchain-developer-summit.splashthat.com/]
By the time this goes out, Max will have given a talk on using Rust to build smart contracts at RustLab in Florence, Italy
Illia presented a workshop to the Denver blockchain community
Illia is back from China, and will be back in China again presenting at a Proof of Stake conference on July 10th
WRITING AND CONTENT
We had a relatively light couple of weeks publishing content. You can check out these new whiteboard series videos and a tour of the economics model we’re implementing.
Augur whiteboard:https://youtu.be/kpW08CiSK9g
NEAR economics design:https://youtu.be/65CKc39lNTw
FOAM whiteboard:https://youtu.be/5L-XbpC4kY0
ENGINEERING HIGHLIGHTS
We’ve had 39 PRs across 10 repos and 10 authors in the last two weeks. Featured repos: nearcore, nearlib, near-shell, and near-wallet. Don’t forget, it’s all open source.
Recently streamlined our release process
App Layer
Updates across all libraries for Nightshade merge
Added advanced access keys in core
near-shell
Updated syntax
Wallet
Bug fixes and UI improvements
Blockchain
First version of staking is complete and currently being tested
Deployed test version Nightshade, our new model consensus
Chunks for sharding are underway in a ~1800 line PR
Proper validators tracking and rotation between epochs (commit).
Shard chunks proper signature and merkle proofs validation (commit)
Tests for cross-shard transactions (commit)
HOW YOU CAN GET INVOLVED
Join us! If you want to work with one of the most talented teams in the world right now to solve incredibly hard problems, check out our careers page for openings. And tell your friends ?
Learn more about NEAR in The Beginner’s Guide to NEAR Protocol. Stay up to date with what we’re building by following us on Twitter for updates, joining the conversation on Discord and subscribing to our newsletter to receive updates right to your inbox.
Reminder: you can help out significantly by adding your thoughts in our 2-minute Developer Survey.
https://upscri.be/633436/ |
---
description: All you need to know about funding and grants on NEAR.
title: Funding
sidebar_position: 2
---
For support guidance with your existing project, please fill out this [Project Support Form](https://airtable.com/shrQk2yWacIj2QA3E).
:::info on this page
* Details on MetaWeb, a venture fund focused on early-stage crypto projects in the NEAR ecosystem
* Backer Network, a global network of nearly 300 VCs, angels, and mentors that provide ecosystem support.
* GTM/Event Funding opportunities and outlines the process to apply for these grants.
:::
## MetaWeb

Metaweb is a global venture fund focused on early stage crypto projects. In particular, Metaweb specializes in projects and business building within the NEAR ecosystem (NEAR-native, Aurora, Octopus, and more in the future).
Visit: [https://www.metaweb.vc/](https://www.metaweb.vc/)
## Backer Network
NEAR Foundation’s Backer Network is a global and diverse network of nearly 300 VCs, angels, advisors, and mentors with a common goal of externally accelerating the growth of the NEAR ecosystem. These backers represent over $20 billion in capital and provide a range of ecosystem support from external capital for fundraising, liquidity providing, advisory services, mentorship, and more.
If you are a project getting ready to raise and looking to tap into the NEAR backer network, please [complete the following form](https://nearprotocol1001.typeform.com/nearvcnetwork?typeform-source=www.google.com) to ensure the details are captured by Corey Pigott, Strategic Funding Manager at NEAR, who will be in touch. If you are a VC/Fund and would like to subscribe to our monthly newsletter to stay up to date on the NEAR ecosystem, please subscribe to the [NF Key Contributors Newsletter](https://near.us14.list-manage.com/subscribe?u=faedf5dec8739fb92e05b4131&id=cdc7be7d09).
## GTM/Event Funding
If you are planning a GTM push or looking to host an event in partnership with NEAR - a side event alongside a major crypto event, a launch event, etc. - and want to explore funding opportunities from NEAR Foundation to support this, please follow the workflow outlined below:
*Ideally, please try to complete and submit with 4 weeks overhead to allow for review*
1. Submit the [Ecosystem Marketing Grants application](https://airtable.com/shrm92EDb6ydLrSxr) form.
2. Create a copy of the [Event Playbook Toolkit](https://docs.google.com/presentation/d/1MysTwrdWgwd7DdEGD-O6CCIwZZ68YxpZWIqzba3k0jk/edit#slide=id.g13072457ef4_0_0), complete the sections that are relevant to your event/GTM push, and email the copy to events@near.foundation
3. Upon approval you will receive an email follow up from the grants team with a milestone form to complete for the funding, as well as an invoice request
4. After the event, please complete the [post-event retro form](https://docs.google.com/document/d/1wGYvxcEIgocgj32NnRnnfCycCQFNKp3iwvfThKwp73E/edit) for the remainder of the funding.
|
NEAR Weekly On Chain Data Report: December 23
NEAR FOUNDATION
December 23, 2022
Transparency continues to be a core focus of the NEAR Fondation, so every week it will publish data to assess the health of the ecosystem. This is to aid the NEAR community in understanding the current state of NEAR across key metrics. The Weekly On Chain Data Report will also appear at the top of quarterly reports as well as monthly funding reports.
Read the Quarterly Transparency Reports.
Read the Q3 Transparency Report
Read Last week’s On-Chain Data Report.
The Importance of Transparency
The NEAR Foundation has been committed to transparency since inception. It values openness to all stakeholders, including investors, builders, and creators. The Foundation’s focus on openness to the entire community will always be a core tenet.
The Foundation values the community’s continued support and feedback as it publishes these reports. It’s part of the Foundation’s ongoing response to the community to provide as much transparency as possible.
New Accounts and Active Accounts
Daily new account (or new wallet) creation ended the week at around 17,000 per day. This is a slight decline from last week, which ended with 20,000 daily new accounts. The peak for this week was logged on December 20th when 19,000 new wallets were created. Put into context, roughly 14,300 wallets were being created daily at the start of December, making it a positive month for new accounts.
If a NEAR wallet makes an on-chain transaction, it’s counted in the Daily Number of Active Accounts metric. This past week, Daily Active Accounts ranged between 39,266 on December 18th to 46,235 on the 19th of the month. This is still well above August and September’s numbers, where Daily Active Accounts hovered around 10,000 with a Q4 2022 high of 183,000 accounts active logged on September 14.
New Contracts and Active Contracts
Smart contracts built on the NEAR blockchain are programs that automatically execute when certain parameters are met. By examining the Daily Number of New Contracts created, the community can get a better sense of how healthy the ecosystem is and its growth as well.
The more new smart contracts are being actively executed, the more projects and users are engaging with NEAR. There was a substantial increase in the number of daily new contracts to end the week, climbing from 28 on the 17th to 46 by the 21st. This high of the last 7 days was 63 on the 16th, and the low was 26 on December 18th.
Daily Number of Active Contracts measures contracts that were executed within a 24-hour time span. This ranged from a high of 797 on December 15th to a low of 535 on the 18th. These numbers are still well above the beginning of the year, where there were roughly between 200 to 400 daily active contracts.
Used Gas and Gas Fee
Gas Fees are the costs associated with conducting transactions on the NEAR network and blockchain. Validators for the network are compensated using Gas Fees for their services, incentivizing validators needed to secure the network.
Over the last week, Used Gas on the NEAR network (or PetaGas) declined slightly from 7,489 on the 15th, ending the week at 6,226. This is fairly consistent with the entire month of December, indicating that individuals are on the network and transacting regularly.
During this past week, there has been a slight drop in the Gas Fee, which correlates with a drop in Used Gas. On December 18th, the Gas Fee was measured at 570, before peaking to 676 the following day. The rest of this week has hovered around 622.
Daily Transactions
Finally, the Daily Number of Transactions shows how many times the blockchain logged a transaction over the past week. This week’s figures indicate a slight decrease from 454,757 on the 16th of December to 332,708 on the 21st.
These reports are generated every week and published on Friday. |
NEAR at Consensus Highlights
NEAR FOUNDATION
April 27, 2023
NEAR has a monolithic mega-booth at Consensus 2023, where there have been a number of awesome NEAR announcements, talks, and events, including the big news out: the Blockchain Operating System is now live on Near.org and the NEAR Horizon accelerator is open for business. If you haven’t stopped by yet to meet our NEAR experts or are unable to attend, we’ve got you covered with all of the highlights.
Let’s take a look at everything NEAR at Consensus, including the BOS, NEAR Horizon, a submissions call for the latest edition of Women in Web3, and much more! And stay tuned for more NEAR at Consensus highlights over the next few days.
The BOS is now live on Near.org
On April 26th at Consensus, NEAR co-founder and Pagoda CEO Illia Polosukhin and Pagoda Chief Product Officer Alex Chiocchi announced that the Blockchain Operating System (BOS) — a real alternative to the Operating Systems of centralized platforms — is now live at near.org.
As a blockchain operating system for an open web, the BOS is accessible to everyone, not just web3 natives. And it makes Web3 and Web2 easier than ever to access and navigate for users and developers alike. With the BOS, you no longer have to choose between decentralization and discoverability. Devs and users get the best of both worlds, whether they are new to Web3 experiences and want to play around, or developers looking to build an open web.
Read the full BOS announcement here.
NEAR Horizon: A revolutionary accelerator for Web3 startups
NEAR Foundation also announced the launch of Near Horizon, a Web3 accelerator program, on the 26th from Consensus. Built on the Blockchain Operating System (BOS), Horizon features a revolutionary marketplace where Web3 startups can find the support they need for building on Near and cross-chain platforms.
Together with partners Dragonfly, Pantera, Decasonc, Blockchange, Fabric Ventures, dLab, Hashed, and Factomind, and Outlier Ventures, Near Horizon is on a mission to empower founding teams with the tools and support they require to accelerate the development of sustainable, profitable, innovative startups on Near.
Near Horizon’s robust network of support is a game-changer for Near builders, offering new opportunities and resources to propel the best minds in the Near ecosystem to success.
Read the full NEAR Horizon announcement here.
NEAR opens submissions for Women in Web3 Changemakers 2023
Following its hugely successful launch a year ago, Women in Web3 is back! On Day 2 of Consensus, NEAR Foundation announced the return of its global Women in Web3 Changemakers list.
Nominations are now open for 2023! Designed to showcase the talented and socially mindful women who make up the Web3 ecosystem, the initiative will once again spotlight ten exceptional leaders across the international sector and the amazing, sustainable work they do.
Members of the Web3 community — both men and women — are welcome to nominate and submit the names of female colleagues who they feel should be recognized for their exemplary contribution to Web3. Women who work in Web3 can also nominate themselves.
Each nominee should meet the following criteria, which NEAR uses to define impact:
Inclusion – driving ideas that are good for society, sustainable, and socially impactful.
Influence – in the community and among peers.
Innovation – contributing to interesting and socially impactful projects at work or independently.
The top ten Changemakers will be decided by you — the community!
Important Dates for Submissions and Voting
More details on the voting process will be announced shortly. But here are some important dates.
June 1, 2023 — Nominations Deadline
June 5, 2023 — Voting Begins
June 19, 2023 (Midnight) — Voting Ends
June 29, 2023 — 10 Changemakers for 2023 will be announced
Those selected will be included in the Women in Web3 Changemakers list, which will be shared with the press and the global community. NEAR will also feature the winners in a special series of video interviews that will air across NEAR’s social platforms and distribution network to share each award-winner’s story and contributions to Web3. Founders of Web3 companies that have made the list will also have the opportunity to meet with investors and pitch for funding at the event.
Google Cloud Unveils Web3 Startup Program
Google Cloud recently made a splash in the Web3 space by announcing its Web3 Startup program via a Twitter thread during Consensus. Catering to seed and Series A projects and startups, the program offers up to $200K in cloud credits, and access to technical training. Recipients also receive partner perks including discounts on Nansen AI products and platform credits from Alchemy, and Thirdweb’s gasless contract deployment, support, and co-marketing opportunities.
Google’s support of Web3 will benefit founders building on the Blockchain Operating System (BOS) and those partnering with the recently launched NEAR Horizon, an accelerator revolutionizing how founders are supported in Web3. NEAR Horizon will enable founding teams to scale their project with the support they need, increasing the number of founding teams building great products with real-world value on the NEAR Protocol.
To apply, visit the application portal and explore more information on the official website.
Here’s how to get involved:
Founders: Join NEAR Horizon here
Accelerator Programmes: Register your interest in a partnership here
Backers: Register your interest in partnering here
NEARCON is Returning to Lisbon and NEAR APAC is Headed to HCMC, Vietnam!
Today at Consensus, NEAR Foundation announced that NEARCON will take place in Lisbon, Portugal on November 7 – 10, 2023. In an effort to globally scale and create more accessible events, the NEAR Foundation also announced that NEAR APAC will take place in Ho Chi Minh City, Vietnam on September 8 – 12, 2023. Both events will gather an audience of builders, Web2 and Web3 entrepreneurs, industry professionals, investors, and creators from all over the world.
NEAR APAC will be the NEAR Ecosystem’s first and largest event in the APAC region. NEAR APAC delivers a world-class lineup of speakers to share latest APAC developments, unlimited potential of the blockchain industry, regional market insights, Web3 hacker festival, and insightful events for networking opportunities with local communities and partners. Head to https://nearapac.org/ on May 15th to secure your NEAR APAC tickets!
NEARCON, the NEAR Ecosystem’s Annual flagship conference is headed back to Lisbon. The Mainnet anniversary of the protocol’s launch and the biggest announcements from the Ecosystem will be featured at NEARCON. Hear from some of the most iconic names in Web3 speak on Technical updates, Web2 and Web3 trends, the latest on Governance and Regulation, Arts and Culture, and much more.
This year the conference will take over two venues: Convento De Beato and Armazem Lisboa. Convento de Beato will host the core conference and will feature two larger stages whereas Armazem Lisboa will be Developers HQ where hackers will build all day. Both venues are just a 10 minute walk from each other. Mark your calendars because on June 1st NEARCON tickets will go live on nearcon.org!
See you in HCMC and Lisbon this Fall!
Updates from around the NEAR Ecosystem
Mailchain brings encrypted communication to NEAR-based dApps
Mailchain, an open source, multi-chain communication layer for Web3, announced integration with NEAR. Now, NEAR developers can send encrypted messages between blockchain wallet addresses with simple, unified inbox to view and send messages and keep track of on-chain activity.
NEAR’s vision has always been to onboard the next billion users to the Open Web. With Mailchain, these next billion users can communicate natively with one another, regardless of blockchain.
Mailchain’s integration with NEAR is a significant step towards strengthening the interoperability mission of NEAR’s blockchain operating system (BOS). NEAR’s BOS and Mailchain are both chain-agnostic, prioritize usability and security, and together make Web3 more accessible and interoperable for users.
“Our vision aligns perfectly with NEAR’s–to onboard the next billion users,” said Tim Boeckmann, CEO of Mailchain. “When building Mailchain, we knew these next billion users would want to communicate with what mattered most to them, their blockchain identities. Mailchain’s secure communication protocol makes this a reality. We believe that this integration is a big step forwards for the developers who are building the next generation of the Internet.”
XP Network brings cross-chain NFT bridge to NEAR
During Consensus, the XP Network, a cross chain NFT bridge, went live on NEAR mainnet. XP Network allows NFT holders to transfer their NFTs to any other supported chain. XP Network recently showcased their cross-chain NFT bridge by transferring a BSC NFT over to Near via the XP Network bridge, and selling it on Mintbase.
Now, NFT marketplaces built on NEAR Protocol like Paras, Berry Club, Mintbase, Flux, Few & Far can list the most promising NFT collections from almost 30 other chains. XP Network’s integration will supercharge both arts and collectibles, as well as Web3 gaming on NEAR, with players now being able to buy and trade NFTs in-game across chains.
kuutamo and Lattitude.sh give NEAR validators access to premium infrastructure
From Consensus, Kuutamo announced its partnership with enterprise-grade infrastructure provider Lattitude.sh, which will give developers and current NEAR validators more flexibility and control. NEAR validators using both kuutamo and Latitude can now access premium infrastructure at a limited promotional price, or apply for the kuutamo x Latitude Program.
A venture-backed technology company, Kuutamo offers a resilient and user-friendly node infrastructure, designed for the peer-to-peer economy. kuutamo launched the world’s first high availability node for the NEAR Protocol, giving users full control and flexibility (no lock-in effect) to execute their desired cloud strategies — differentiating itself from most large public cloud providers in the process.
Latitude is an emerging global leader, offering access to enterprise-grade infrastructure in the world’s most advanced data centers. In its partnership with kuutamo, Latitude is expanding its offering to developers and NEAR validators. Latitude already supports other leading blockchains and Web3 companies such as Solana, Ankr, and Riot Games.
WOMBI brings Web2-style analytics to NEAR
During Consensus, Wombi — a Web3 attribution platform — announced that it is now live on NEAR. With an aim to be the Google Analytics of Web3, Wombi helps decentralized app developers by connecting Web2 and Web3 funnels, so they can instantly see which marketing channels are bringing them the most impactful users.
Wombi is now publicly available to all dApps fully live or building on the NEAR Blockchain Operating System or Protocol. All developers get free product and marketing analytics, making NEAR the first Layer 1 with a live attribution product. |
---
sidebar_position: 1
sidebar_label: Introduction
pagination_label: "Promises: Introduction"
---
# Promises
Transactions can be sent asynchronously from a contract through a [`Promise`](https://docs.rs/near-sdk/latest/near_sdk/struct.Promise.html). Like Promises in many programming languages, these will cause code to be executed in the future. In the case of NEAR, this "in the future" means a transaction to be executed _in the next block_ (or thereabouts), rather than in the same block as the original function call.
You can implement any cross-contract workflow using Promises; they inhabit a middle-ground between the high-level and low-level approaches discussed in [the last section](../cross-contract/callbacks.md). See the full Promise docs, linked above, for details.
However, there are a few situations where Promises are uniquely capable, since these situations don't involve making function calls:
* Sending $NEAR
* Creating accounts
* Deploying contracts
:::info Why wait?
Why not do these things synchronously, in the same block when the function is called? Why does NEAR require a `Promise` for sending tokens, or creating an account, or deploying a contract?
They need to be scheduled in separate blocks since sender and receiver accounts can be on different shards, and cross-shard communication happens across blocks by passing receipts (you can think of receipts in NEAR as "internal transactions"). You can see these receipts being passed from block to block [in NEAR Explorer](https://nearblocks.io/txns/36n3tBNiF497Tm9mijEpsCUvejL8mBYF1CEWthCnY8FV).
::: |
---
title: Enterprise Solutions
description: Overview of how NEAR BOS can be used for Enterprise Solutions
sidebar_position: 8
---
# Enterprise Solutions
Coming soon! |
---
id: reentrancy
title: Reentrancy Attacks
---
Between a cross-contract call and its callback **any method of your contract can be executed**. Not taking this into account is one of the main sources of exploits. It is so common that it has its own name: **reentrancy attacks**.
Always make sure to keep your state in a consistent state after a method finishes executing. Assume that:
- Any method could be executed between a method execution and its callback.
- The same method could be executed again before the callback kicks in.
---
### Example
Imagine that we develop a `deposit_and_stake` with the following **wrong logic**: (1) The user sends us money, (2) we add it to its balance, (3) we try to stake it in a validator, (4) if the staking fails, we remove the balance in the callback. Then, a user could schedule a call to withdraw between (2) and (4), and, if the staking failed, we would send money twice to the user.

*Between a cross-contract call and the callback anything could happen*
Luckily for us the solution is rather simple. Instead of immediately adding the money to our user’s balance, we wait until the callback. There we check, and if the staking went well, then we add it to their balance.
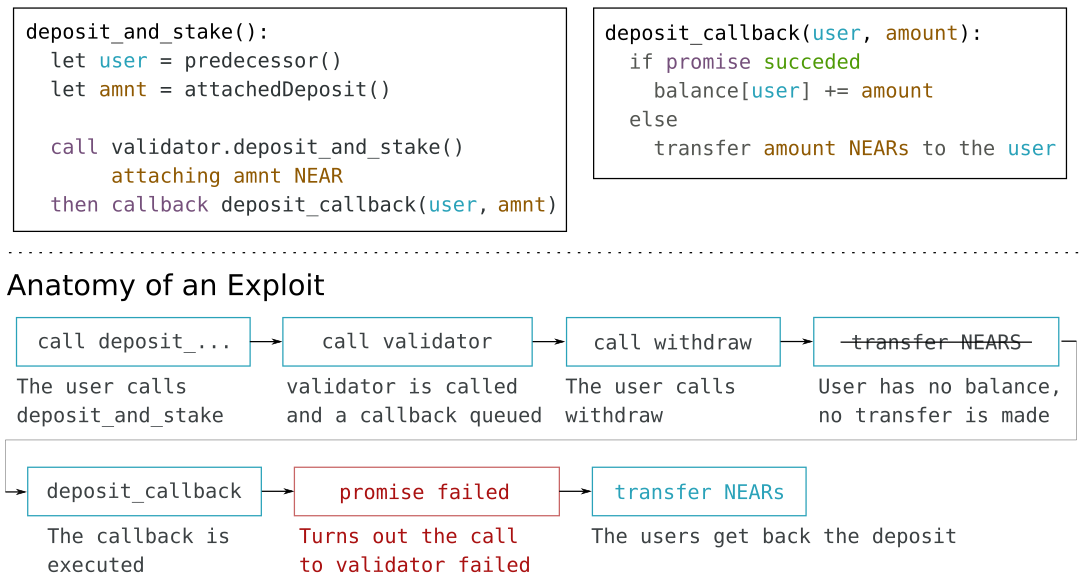
*Correct way to handle deposits in a cross-contract call* |
NEARCON 2022: Highlights, Talks and Videos
COMMUNITY
September 20, 2022
Missed NEARCON 2022? We’ve got you covered with content from the four conference stages, as well as a dizzying host of awesome announcements.
Here’s a sizzle to get you primed for all that happened at this year’s NEARCON. A NEARCON 2022 Dropbox folder has also been set up so everyone can peruse, download, and share all of the photos from the conference. There is also a NEARCON 2022 YouTube video playlist worth checking out.
NEARCON Day 1: Year in Review, Regulatory Frameworks, Brave Fireside Chat, and More
Day One kicked off with a slew of exciting speakers, sessions, and announcements. It was headlined by notable names from an array of industries and backgrounds, from JavaScript inventor Brandon Eiche to renowned crypto legal expert Sheila Warren.
From NEAR’s first ever transparency report to the announcement of a new Sports and Entertainment fund, here’s all the big news from Day One of NEARCON.
Ecosystem Announcements
Few and Far, a next-generation NFT marketplace built on NEAR, won a grant to advance NFTs across the ecosystem.
NEAR unveiled the eleven winners of the Women in Web3 Changemakers competition to spotlight the achievement of women in Web3 and to showcase female role models in the ecosystem.
Tether, the first and most widely used stablecoin (“USDT”), launched on NEAR.
Sender Wallet raised US$4.5 Million in a private round led by Pantera Capital.
NEAR Digital Collective (NDC) will help decentralize NEAR’s ecosystem governance and move decision-making on-chain to make community more resilient, transparent, and fair.
Phase 1 of NEAR’s Nightshade sharding was unveiled, making NEAR even more decentralized and scalable.
In a new Entertainment Fund, NEAR is backing a new $100m VC Fund and Lab focused on Web3’s evolution of culture, media, and entertainment
The Fireblocks integration on NEAR went live.
Day 1 Videos
Layer 1 Stage
Nightshade Stage
Doomslug Stage
NEAR Year in Review and Transparency Report
NEAR Foundation CEO Marieke Flament and NEAR Co-founder Illia Polosukhin began the day by welcoming attendees on the Layer 1 Stage and delving into how NEAR is building beyond the hype through 2022 and beyond.
“We’re extremely excited about the diversity of attendees and over 220 speakers from so many different verticals and industries,” said Flament. “And we’ll continue to build upon our core vision of onboarding the next one billion users onto Web3 by helping the ecosystem build things that people can use.”
Flament returned solo to the stage for the “NEAR Year in Review,” recapping key milestones in the ecosystem over the last 12 months and unveiling NEAR’s first ever Transparency Report. Flament communicated top data from the report, including how the NEAR Grants fund had reached over $800 million in capital with over $540 million deployed within the ecosystem. Among the key sectors of grant recipients were Startup Funds, Regional Hubs, NFTs, Gaming and & Entertainment and DAOs.
Watch the full talk.
Bridging the Gap Between Web2 and Web3
Onboarding the next billion users onto Web3 requires an efficient tech stack and rich ecosystem of useful decentralized apps (dApps) and services, a topic that James Tromans from Google Cloud took to the Layer 1 Stage to address. While Tromans championed Web3, he also pointed out the Web2 services can play a role in easing the transition for developers.
“We need to make building in Web3 faster, better, and easier,” Tromans said. “For now, we can bring the best of Web2 services like Google Cloud to Web3 so that not everything has to be built from scratch.”
Tromans was then joined by a panel moderated by Emily Parker from Coindesk entitled “Bridge Builders, Why We Need Web 2.5.” In addition to Parker and Tromans, Susie Batt from Opera Browser and Satish Ramakrishan of BitGo contributed their opinions on the concept of a “Web 2.5” that could enable a more seamless transition to Web3.
Watch the full talk.
Shelia Warren discusses Web3 regulatory frameworks
One of the biggest names headlining day one was Sheila Warren, crypto legal expert and CEO of the Crypto Council for Innovation. Warren took the Layer One stage during the afternoon to help attendees wade through the complex and controversial topics of regulation, governance, and legal frameworks in crypto and Web3.
“Who regulates crypto?” Warren posited to begin. “There is no definitive answer other than it’s complicated. It depends how projects are classified and certain power dynamics between the industry and regulators. What we need is a regulatory policy framework that addresses multiple aspects of decentralization.”
Warren added that regulation is not all bad and could have a beneficial effect on the space if done in an intelligent and collaborative manner.
Watch the full talk.
The next year on NEAR and Brave Fireside Chat
Looking ahead, NEAR Co-founder Illia Polosukhin took the Layer 1 stage to offer his sentiments on the year ahead for the NEAR Foundation, protocol, and ecosystem. Polosukhin predicted a steady increase in both ecosystem funding and projects, with over 800 projects and growing, according to AwesomeNEAR.
“I’m very excited about next year as we’ll continue to build out a wide range of developer tooling that will facilitate the next generation of NEAR apps,” said Polosukhin. “We now have all the necessary primitives – like Paras for NFTs and AstroDAO for DAOs – that the NEAR ecosystem can now become more composable with richer experiences.”
Watch the full talk.
Polosukhin then welcomed Brave founder Brandon Eiche to the stage for a fireside chat. Eiche is also the inventor of the JavaScript programming language, and the two discussed a wide array of topics including NEAR’s recently launched JavaScript SDK.
“Everything NEAR is doing feels like one of my children,” Eiche said. “On-chain JavaScript is simply a dream, and now so many developers can build on-chain without learning another language.”
Watch the full talk.
NEARCON Day 2: Headlined by Messari, Armored Kingdom, and SailGP
Most anyone at Day Two of NEARCON could attest to the level of excitement and momentum for the entire NEAR ecosystem. And if there’s one thing that Day Two showcased, it’s that Web3 and NEAR are well along the path of mass adoption. From sports to social media and entertainment, real-world projects building on NEAR stepped forward in a big way on Day Two.
Session attendees came away with big updates and predictions for NEAR ecosystem partners like Sweatcoin and SailGP, not to mention a special appearance and workshop for the creators of Armored Kingdom. Everyone in the building also saw that the Hackathon was in full swing, with presentations and judging right around the corner.
Ecosystem Announcements
New NEAR Regional Hubs launched in India and Vietnam to support Web3 innovation, education, and talent.
Pagoda Console lets devs create without limits from an ad hoc toolkit, a one-stop tool shop that gives devs all they need to build Web3 apps.
Switchboard goes multichain with NEAR to give developers the best tools and data to build their apps.
Fayyr launched first NFT Marketplace for Social Impact Organizations on NEAR.
Chainstack, a leading blockchain managed services provider, launched support for NEAR on its platform.
Contented Media Group (CMG) received a Near Foundation grant to launch a Web3 platform where brands can interact with content creators.
Bitstamp listed NEAR token on its exchange.
Day 2 Videos
Layer 1 Stage
Nightshade Stage
Doomslug Stage
Proof of Sound Stage
Ryan Selkis of Messari on navigating crypto cycles
Things started off with one of the biggest names at NEARCON, as Messari co-founder Ryan Selkis joined NEAR CEO Marieke Flament to discuss where we are in the current crypto cycle, building in a downturn, and how to navigate the future. Selkis was well-positioned to speak on the subject, having lived through multiple peaks and valleys of blockchain markets and adoption.
“We’re in the early innings of a long-term game,” Selkis told Flament. “Centralized institutions are weaker than ever and there’s transformational potential in a user-owned economy, empowering individuals and building more resilient systems.”
“This is the part of the cycle where protocols will begin to cross the chasm from MVP to mass adoption,” posited Selkis. “And now is the time to think long term about things like how the Web3 tech stack will develop and what a potential cross-chain future looks like.”
Watch the full talk.
The SWEAT Economy gets NEARCON moving
Another major highlight of Day Two took place on the Layer One stage as Oleg Fomenko, co-founder of Sweatcoin, explained how the SWEAT economy was born, why the NEAR blockchain is playing a critical role, and where the move-to-earn economy is heading.
“We are literally witnessing the birth of the movement economy,” Fomenko exclaimed. “We created Sweatcoin in 2016 with that name because we assumed it would be on the blockchain. Now we’re number one on the App Store in over 66 countries with over 120 million users.”
Fomenko also recalled that in the early days of Sweatcoin, the Ethereum network simply couldn’t handle the number of transactions with adequate speed to truly bring his vision of move-to-earn to life.
“But when we found NEAR, it was a perfect match in terms of both vision and mission,” said Fomenko. “A fast, low-cost blockchain along with an incredible team is helping us push the movement economy forward and help users live healthier lifestyles.”
Watch the full talk.
SailGP + NEAR Have the wind at their backs
Piggybacking on Day One’s announcement of the NEAR Foundation’s new Sports and Entertainment fund, NEAR CEO Marieke Flament returned to the stage to welcome SailGP’s CEO Russell Coutts and CTO Warren Jones.
“SailGP as a brand and product is built on both being exciting and data driven,” said Coutts. “Our boats generate billions of data points per day that we use to improve the fan experience. We’ve always been technology focused, which is why working with NEAR to integrate Web3 and the blockchain makes so much sense.”
Flament added that one of the most exciting aspects to the partnership is how NEAR is currently helping SailGP facilitate a racing team being owned and operated by a DAO. Coutts also expressed this excitement, painting a picture of endless possibilities with how the DAO might choose to operate and increase fan engagement.
“The DAO might decide that certain token holders or the most engaged members can actually talk to athletes in the midst of a competition,” said Coutts. “Other things like special rewards for members or participating in team selection can also potentially be implemented. It’s all up to the DAO, which is what makes this initiative so special.”
Watch the full talk.
Armored Kingdom gives a lesson in storytelling
One of the most anticipated gaming and metaverse projects finally touched down on Day Two of NEARCON. Armored Kingdom creators Lisa and Hugh Sterbakov were joined by writer Brian Turner to deconstruct the process of building a story arc, core elements to great storytelling, and what to expect from Armored Kingdom on NEAR.
“Armored Kingdom is not just a comic book or metaverse game,” said Lisa. “It’s an entire multimedia platform built where fans can participate and co-create. And we’ll be rolling out an animated series to go along with it.”
“Armored Kingdom is our playground,” Turner said. “The world is based on an endless conflict between four major tribes on an alien planet. There are religious undertones as well as a battle for a scarce resource – Armorite – with which very powerful weapons are made from.”
Lisa capped off the session by mentioning that building Armored Kingdom on NEAR is allowing the team to build features and functions into the game easily due to the tech stack, and in the end, fans can put as much or as little time in as they want.
“Web3 is allowing us to create communities and encourage participation that Web2 never has,” she said. “We’re currently receiving fan art submissions through our Discord community that have already made their way into the comic book.”
Watch the full talk.
NEARCON Day 3: Circle, AstroDAO, and NEAR Digital Collective
The biggest NEARCON to date came to a close on Day Three with a slew of insightful speakers, interactive panels, and an unexpected announcement. Big names from organizations and projects like Circle, NearPay, and A16z helped make the conclusion of NEARCON 2022 feel extremely meaningful and memorable.
Attendees got a glimpse at how DAOs work under the hood, where NEAR fits in with the ever-evolving fintech and payments space, and how builders should approach governance in a crypto winter. And then there was the unveiling of the NEAR Digital Collective, an ambitious leap in decentralizing and enhancing governance on the NEAR network.
Ecosystem Announcements
SWEAT and NEAR partner to enable the masses to ‘walk into crypto.’
OnMachina to provide native large-scale storage system to dApps and services building on NEAR, creating capacity to support billions of users.
Day 3 Videos
Layer 1 Stage
Nightshade Stage
Doomslug Stage
Proof of Sound Stage
Spotlight on Crypto Payments and Fintech
Day Three began with a meeting of the minds between NEAR CEO Marieke Flament and Jeremy Allaire, CEO of Circle. The pair started a morning of talks and discussions around crypto innovation, payments, and broader implications for governments and fintech.
“You couldn’t have predicted the amount of innovation that’s taken place over the past decade and the millions of developers now on the blockchain,” Allaire from Circle said. “And now that we have the base layer technology in place for programmable money, along with decreasing costs of transactions, the stage is really set for exponential growth.”
Flament then asked Allaire about his perspective on the role that stablecoins and central bank digital currencies (CBDC) could play in the future of a blockchain and crypto-powered global financial ecosystem.
“What we really want to see is a composable system that resembles the software-powered internet,” Allair continued. “There’s certainly room for various stablecoins and CBDCs, as long as they continue the inertia towards self-sovereign commerce.”
The duo was followed by a panel entitled “Crypto Natives vs. Fintechs for the Future of Payments,” including notable names from Checkout.com, Roketo, and NearPay. The group discussed a range of topics, from making crypto payments more seamless for individuals and onboarding small businesses to the blockchain.
Watch the full talk.
Deep Dive into DAOs and Governance
The afternoon on the Layer 1 Stage was filled with opinions and insights about Decentralized Autonomous Organizations (DAOs), how they function, and the role of DAOs in the future of Web3 and society at large. In a panel about How DAOs Get Work Done, founders and leaders from AstroDAO, The Kin DAO, and CornerstoneDAO all offered unique perspectives.
“We created Kin DAO for the purposes of collaboratively owning physical land to create self-sustaining food systems and micro economies,” said Adrian Bello, founder of Kin DAO and Primordia DAO. “We’re practicing this decentralized governance in person and rehabilitating our relationship with Earth.”
Bello went on to explain that her new project, Primorida DAO, has the goal of onboarding as many DAOs as possible from excluded communities onto NEAR. That’s in large part because of the NEAR ecosystem’s focus on user-friendly DAO tooling and community building through AstroDAO.
“AstroDAO is our home for everything,” Bello continued. “Other chains can be somewhat hard to get into. But AstroDAO is accessible and has a low barrier to entry. We can reference decisions made on-chain and governance is therefore always transparent.”
Jordan Gray, the founder of AstroDAO, also explained how his project is focused on letting everyday users harness the power of DAOs. Smart contracts, APIs, and governance protocols all exist “under the hood” of AstroDAO, making it easier for both users and developers to create, engage with, and operate DAOs.
“DAOs are going to rock the business world in a way that we haven’t seen since the spreadsheet,” Gray predicts. “We’re on the cusp of a work revolution. And if we figure out new ways to incentivize voting, we might even fix democracy.”
Watch the full talk.
Introducing the NEAR Digital Collective for Governance
Day Three of NEARCON concluded with one of the most exciting announcements of the entire conference. NEAR co-founder Illia Polosukhin and Harry Tafolla of Cheddar announced the creation of the NEAR Digital Collective (NDC), an ambitious initiative to further decentralize NEAR governance and create a more robust, resilient network.
“With the NEAR Digital Collective, we’re striving for a truly autonomous ecosystem,” Polosukhin said. “We need to do this on the people’s level, where we’re all accountable and responsible and systems are not chaotic or toxic.”
Polosukhin went on to explain that the NDC governance framework is akin to drafting the constitution of a newly formed nation, with core community members like Tafolla leading the charge to formulate, define, and implement NEAR governance from here on out.
Polosukhin said that the NDC is a major step in addressing the needs of all stakeholders within the NEAR ecosystem, from users and DAOs to node validators and wallet developers. The NDC will also be dynamic and allow for constant updates and upgrades in governance frameworks and processes.
Watch the full talk.
Hackathon winner and wrapping up NEARCON
Flament then took the stage along with Polosukhin to wrap up NEARCON 2022, highlighting that almost 3,000 attendees from 176 countries contributed to making this year’s gathering such a rousing success. In addition, 38% of all attendees were women, and NEAR handed out the first-ever Women in Web3 Changemakers awards for female role models in the space.
Polosukhin also thanked the 500+ hackers who participated in the NEARCON 2022 Hackathon. Out of the 98 submissions, the winner chosen was Pomegrant, a browser plugin that allows users to eliminate ads and donate directly to content creators using crypto.
“If there’s something that we hope you’re taking away from these past three days, it’s without limits,” Flament concluded. “We hope with NEAR you see that you can truly create without limits and realize whatever dreams and visions that you have for both your project and society. So let’s continue to create without limits, build beyond the hype, and get to one billion users!”
Watch the full talk.
Thanks again to all who attended, contributed to, and engaged with NEARCON 2022. A big round of applause for the entire NEAR ecosystem and we’ll see you again at NEARCON 2023!
|
---
id: prototyping
sidebar_label: Rapid Prototyping
title: "Upgrading Contracts: Rapid Prototyping"
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
# Rapid Prototyping
When you change the interface of a contract and re-deploy it, you may see this error:
Cannot deserialize the contract state.
### Why does this happen?
When your contract is executed, the NEAR Runtime reads the serialized state from disk and attempts to load it using current contract code. When your code changes but the serialized state stays the same, it can't figure out how to do this.
### How can you avoid such errors?
When you're still in the Research & Development phase, building a prototype and deploying it locally or on [testnet](../../../1.concepts/basics/networks.md), you can just delete all previous contract state when you make a breaking change. See below for a couple ways to do this.
When you're ready to deploy a more stable contract, there are a couple of [production strategies](../../../2.build/2.smart-contracts/release/upgrade.md#migrating-the-state) that will help you update the contract state without deleting it all. And once your contract graduates from "trusted mode" (when maintainers control a [Full Access key](/concepts/protocol/access-keys)) to community-governed mode (no more Full Access keys), you can set up your contract to [upgrade itself](../../../2.build/2.smart-contracts/release/upgrade.md#programmatic-update).
## Rapid Prototyping: Delete Everything All The Time
There are two ways to delete all account state:
1. Deploying on a new account each time
2. Deleting & recreating contract account
For both cases, let's consider the following example.
Let's say you deploy [a JS status message contract](https://github.com/near/near-sdk-js/blob/263c9695ab7bb853ced12886c4b3f8663070d900/examples/src/status-message-collections.js#L10-L42) contract to testnet, then call it with:
<Tabs className="language-tabs" groupId="code-tabs">
<TabItem value="near-cli">
```bash
near call [contract] set_status '{"message": "lol"}' --accountId you.testnet
near view [contract] get_status '{"account_id": "you.testnet"}'
```
</TabItem>
<TabItem value="near-cli-rs">
```bash
near contract call-function as-transaction [contract] set_status json-args '{"message": "lol"}' prepaid-gas '30 TeraGas' attached-deposit '0 NEAR' sign-as you.testnet network-config testnet sign-with-keychain send
near contract call-function as-read-only [contract] get_status text-args '{"account_id": "you.testnet"}' network-config testnet now
```
</TabItem>
</Tabs>
This will return the message that you set with the call to `set_status`, in this case `"lol"`.
At this point the contract is deployed and has some state.
Now let's say you change the contract to store two kinds of data for each account, a status message and a tagline. You can add to the contract code a `LookupMap` for both status message and another one for the tagline, both indexed by the account ID.
You build & deploy the contract again, thinking that maybe because the new `taglines` LookupMap has the same prefix as the old `records` LookupMap (the prefix is `a`, set by `new LookupMap("a"`), the tagline for `you.testnet` should be `"lol"`. But when you `near view` the contract, you get the "Cannot deserialize" message. What to do?
### 1. Deploying on a new account each time
When first getting started with a new project, the fastest way to deploy a contract is [creating an account](../../../4.tools/cli.md#near-create-account) and [deploying the contract](../../../4.tools/cli.md#near-deploy) into it using `NEAR CLI`.
<Tabs className="language-tabs" groupId="code-tabs">
<TabItem value="near-cli">
```bash
near create-account <account-id> --useFaucet
near deploy <account-id> ./path/to/compiled.wasm
```
</TabItem>
<TabItem value="near-cli-rs">
```bash
near account create-account sponsor-by-faucet-service <my-new-dev-account>.testnet autogenerate-new-keypair save-to-keychain network-config testnet create
near contract deploy <my-new-dev-account>.testnet use-file <route_to_wasm> without-init-call network-config testnet sign-with-keychain
```
</TabItem>
</Tabs>
This does a few things:
1. Creates a new testnet account pre-funded with 10N from the faucet
2. Stores the private key for this account in the `~/.near-credentials` folder
3. Deploys your contract code to this account
### 2. Deleting & Recreating Contract Account
Another option to start from scratch is to delete the account and recreate it.
<Tabs className="language-tabs" groupId="code-tabs">
<TabItem value="near-cli">
```bash title="Delete sub-account"
near delete <accountId> <beneficiaryId>
```
</TabItem>
<TabItem value="near-cli-rs">
```bash title="Delete sub-account"
near account delete-account app-name.you.testnet beneficiary you.testnet network-config testnet sign-with-keychain send
```
</TabItem>
</Tabs>
This sends all funds still on the `<accountId>` account to `<beneficiaryId>` and deletes the contract that had been deployed to it, including all contract state.
Now you create the sub-account and deploy to it again using the commands above, and it will have empty state like it did the first time you deployed it.
|
Zero-commitment onboarding to NEAR using Gitpod
COMMUNITY
April 14, 2020
NEAR has discovered a fantastic tool called Gitpod that gives new developers a kick-start, improves the efficacy of community code reviews, and saves resources on a developer’s machine.
With two clicks, a new developer comes to this:
It looks like an integrated development environment (IDE), but… on a web page? Yes, it is. A Docker-based image has been spun up, language/platform versions locked in, and dependencies have been installed automatically. There’s even a Terminal, giving the developer a perfectly-working project ready to poke and prod at. Before we get into more technical details, let’s take a step back.
Motivation
NEAR has a history of carefully choosing focus points for a scalable blockchain. Sharded, proof-of-stake blockchains are still in nascent stages and offer intriguing challenges. The most effective way for the community of contributors (the NEAR Collective) to thrive is twofold: first, to pick the right technological battles, and second, to use great tools when they’re identified. This is a reason why WebAssembly has been the backbone of NEAR’s virtual machine (VM). There are enough puzzles to solve without writing and maintaining a new VM. The same thing goes for writing smart contracts on NEAR. While there are customized bindings, NEAR utilizes reliable languages like Rust and AssemblyScript instead of writing another programming language. NEAR resists the urge to remake peripheral touchpoints of a blockchain system and instead focuses on making the best blockchain with the best tools.
Gitpod is one of those tools discovered recently. The Gitpod website lays it out clearly: Gitpod is a tool allowing developers to launch ready-to-code development environments for your Github projects with a single click. The code at NEAR Protocol lives on Github, where the NEAR Collective in building out the codebase. With a couple of settings, Gitpod integrates beautifully with Github in two ways:
Repository landing page — a Gitpod button opens the repository in the selected branch (typically master) This saves the end-user time and energy that would go into setting up a development environment.
Pull Requests — a Gitpod button appears on pull requests, showing a live version with the given changes. (This is excellent for quality assurance and code review.)
Gitpod in action
Expanding on the first bullet point above, NEAR has launched the new examples page showing various, simple, smart contracts that illustrate different facets of decentralized app (dApp) development. For instance, an example may show basic reading/writing to the blockchain with a Rust contract, or perform a cross-contract call, or create a custom token, or transfer tokens with an escrow system, etc. Using Gitpod, a developer has a frictionless experience getting an example application started. Gitpod uses a tried-and-true online IDE called Theia, which is fully compatible with VS Code extensions.
Gitpod also saves builds of the projects you’ve added to your “Gitpod workspaces” to further speed things up. We’ll not go into all the useful features of Gitpod, but encourage you to check them out.
NEAR has hosted a number of online and in-person hackathons and workshops. (At the time of this writing, we’re very excited about the upcoming Ready Layer One online event taking place May 4-6, 2020.) During in-person workshops, there’s historically been a battle between a shared internet connection and the participants downloading dependencies, tools, virtual machines, Docker images, etc. Gitpod is terrific for this use case as well, offloading the downloads and processing power elsewhere. Again, after a couple of clicks, the end-user finds a turnkey development environment ready to build on NEAR. Gitpod allows for a zero-commitment onboarding for everyone regardless of the operating system, internet speed, administrative permissions, and one’s development environment setup.
Wait, why not just clone a project?
NEAR understands that developers have options and limited time. Every second NEAR can save a developer is precious. That said, here’s a natural progression one might follow to build on NEAR, starting at zero-commitment, to local development, to a full dApp:
Copy/paste commands from the NEAR homepage to build a React app that integrates with the blockchain.
Eager to learn more, visit the examples page, and select an interesting one.
Click the “Open in Gitpod” button.
Follow the instructions in the README.
Sandbox time: change the source, build it again! (See README file for instructions)
Decide this (or another) example is the starting point for a personal or business dApp.
Follow the example page’s link to the Github repository and clone it.
Create a NEAR account using NEAR Wallet (In Gitpod, the NEAR accounts were automatically generated for convenience sake. They have rather dull names, so we typically create a more personalized account for further development.)
Continue local development; possibly even run against a local NEAR blockchain.
Create something you’re proud of, commit it to your own Github (or elsewhere) repository.
Give it a frontend (we have many examples of this) and deploy it! (Again, see README file for instructions)
Keep hacking, and hang out with us on Telegram.
Conclusion / future thoughts
Gitpod is an amazing tool that significantly cuts downtime for two important personas:
Members of the NEAR Collective making contributions to and reviewing code on Github
New developers who can’t/won’t alter their computer’s development environment
There’s untold potential in the Gitpod approach laid out here. Teachers can be introducing blockchain development in classrooms without having to install Rust on every computer. A curious mind can hack away on their first dApp using a public library’s computer with restricted permissions. End users struggling with an error can include a link to a Gitpod snapshot where an error can be reliably reproduced. It will be fascinating to see how else the development/learning lifecycle can benefit from a tool like this, and NEAR is excited to lean into it.
Lastly, dig into a Gitpod example for yourself at https://near.dev.
|
---
sidebar_position: 3
---
# Collections Nesting
## Traditional approach for unique prefixes
Hardcoded prefixes in the constructor using a short one letter prefix that was converted to a vector of bytes.
When using nested collection, the prefix must be constructed manually.
```rust
use near_sdk::borsh::{self, BorshDeserialize, BorshSerialize};
use near_sdk::collections::{UnorderedMap, UnorderedSet};
use near_sdk::{near_bindgen, AccountId};
#[near_bindgen]
#[derive(BorshDeserialize, BorshSerialize)]
pub struct Contract {
pub accounts: UnorderedMap<AccountId, UnorderedSet<String>>,
}
impl Default for Contract {
fn default() -> Self {
Self {
accounts: UnorderedMap::new(b"t"),
}
}
}
#[near_bindgen]
impl Contract {
pub fn get_tokens(&self, account_id: &AccountId) -> Vec<String> {
let tokens = self.accounts.get(account_id).unwrap_or_else(|| {
// Constructing a unique prefix for a nested UnorderedSet from a concatenation
// of a prefix and a hash of the account id.
let prefix: Vec<u8> = [
b"s".as_slice(),
&near_sdk::env::sha256_array(account_id.as_bytes()),
]
.concat();
UnorderedSet::new(prefix)
});
tokens.to_vec()
}
}
```
## Generating unique prefixes for persistent collections
Read more about persistent collections [from this documentation](../contract-structure/collections.md) or from [the Rust docs](https://docs.rs/near-sdk/latest/near_sdk/collections).
Every instance of a persistent collection requires a unique storage prefix.
The prefix is used to generate internal keys to store data in persistent storage.
These internal keys need to be unique to avoid collisions (including collisions with key `STATE`).
When a contract gets complicated, there may be multiple different
collections that are not all part of the main structure, but instead part of a sub-structure or nested collections.
They all need to have unique prefixes.
We can introduce an `enum` for tracking storage prefixes and keys.
And then use borsh serialization to construct a unique prefix for every collection.
It's as efficient as manually constructing them, because with Borsh serialization, an enum only takes one byte.
```rust
use near_sdk::borsh::{self, BorshDeserialize, BorshSerialize};
use near_sdk::collections::{UnorderedMap, UnorderedSet};
use near_sdk::{env, near_bindgen, AccountId, BorshStorageKey, CryptoHash};
#[near_bindgen]
#[derive(BorshDeserialize, BorshSerialize)]
pub struct Contract {
pub accounts: UnorderedMap<AccountId, UnorderedSet<String>>,
}
impl Default for Contract {
fn default() -> Self {
Self {
accounts: UnorderedMap::new(StorageKeys::Accounts),
}
}
}
#[derive(BorshStorageKey, BorshSerialize)]
pub enum StorageKeys {
Accounts,
SubAccount { account_hash: CryptoHash },
}
#[near_bindgen]
impl Contract {
pub fn get_tokens(&self, account_id: &AccountId) -> Vec<String> {
let tokens = self.accounts.get(account_id).unwrap_or_else(|| {
UnorderedSet::new(StorageKeys::SubAccount {
account_hash: env::sha256_array(account_id.as_bytes()),
})
});
tokens.to_vec()
}
}
```
## Error prone patterns
By extension of the error-prone patterns to avoid mentioned in the [collections section](./collections.md#error-prone-patterns), it is important to keep in mind how these bugs can easily be introduced into a contract when using nested collections.
Some issues for more context:
- https://github.com/near/near-sdk-rs/issues/560
- https://github.com/near/near-sdk-rs/issues/703
The following cases are the most commonly encountered bugs that cannot be restricted at the type level:
```rust
use near_sdk::borsh::{self, BorshSerialize};
use near_sdk::collections::{LookupMap, UnorderedSet};
use near_sdk::BorshStorageKey;
#[derive(BorshStorageKey, BorshSerialize)]
pub enum StorageKey {
Root,
Nested(u8),
}
// Bug 1: Nested collection is removed without clearing it's own state.
let mut root: LookupMap<u8, UnorderedSet<String>> = LookupMap::new(StorageKey::Root);
let mut nested = UnorderedSet::new(StorageKey::Nested(1));
nested.insert(&"test".to_string());
root.insert(&1, &nested);
// Remove inserted collection without clearing it's sub-state.
let mut _removed = root.remove(&1).unwrap();
// This line would fix the bug:
// _removed.clear();
// This collection will now be in an inconsistent state if an empty UnorderedSet is put
// in the same entry of `root`.
root.insert(&1, &UnorderedSet::new(StorageKey::Nested(1)));
let n = root.get(&1).unwrap();
assert!(n.is_empty());
assert!(n.contains(&"test".to_string()));
// Bug 2 (only relevant for `near_sdk::collections`, not `near_sdk::store`): Nested
// collection is modified without updating the collection itself in the outer collection.
//
// This is fixed at the type level in `near_sdk::store` because the values are modified
// in-place and guarded by regular Rust borrow-checker rules.
root.insert(&2, &UnorderedSet::new(StorageKey::Nested(2)));
let mut nested = root.get(&2).unwrap();
nested.insert(&"some value".to_string());
// This line would fix the bug:
// root.insert(&2, &nested);
let n = root.get(&2).unwrap();
assert!(n.is_empty());
assert!(n.contains(&"some value".to_string()));
```
|
---
id: tokens
title: Tokens
---
# NEAR Token
This is the native token used in NEAR Protocol.
It has multiple use cases:
- Secures the network through staking
- Provides a unit of account - NEAR is used for processing transactions and storing data
- Serves as a medium of exchange
### Securing the Network
NEAR Protocol is a proof-of-stake (PoS) network, which means that resistance from various attacks comes from staking NEAR.
Staked NEAR represents the decentralized infrastructure of servers that maintain the network and process transactions for applications and users on NEAR.
Rewards for providing this service are received in NEAR.
## Providing a Unit of Account
NEAR is used to price computation and storage on the NEAR infrastructure.
The network charges transaction fees in NEAR to process changes and transactions.
## Medium of Exchange
NEAR is readily available on the protocol level, so it can be used to transfer value between NEAR applications and accounts.
This means that applications can use NEAR to charge for various functions, like access to data or other complex transactions.
Entities can also easily exchange NEAR between each other, without the need for trusted third parties to clear and settle transactions.
For a deeper dive on NEAR Economics: [https://near.org/blog/near-protocol-economics](https://near.org/blog/near-protocol-economics)
For more information about the NEAR token, visit [NEAR Token Supply and Distribution](https://near.org/blog/near-token-supply-and-distribution/) or [Nomicon](https://nomicon.io).
|
---
id: transactions
title: RPC Endpoints
sidebar_label: Transactions
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
The RPC API enables you to send transactions and query their status.
---
## Send transaction {#send-tx}
> Sends transaction.
> Returns the guaranteed execution status and the results the blockchain can provide at the moment.
- method: `send_tx`
- params:
- `signed_tx_base64`: SignedTransaction encoded in base64
- [Optional] `wait_until`: the required minimal execution level. [Read more here](#tx-status-result). The default value is `EXECUTED_OPTIMISTIC`.
Using `send_tx` with `wait_until = NONE` is equal to legacy `broadcast_tx_async` method.
Using `send_tx` with finality `wait_until = EXECUTED_OPTIMISTIC` is equal to legacy `broadcast_tx_commit` method.
Example:
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "send_tx",
"params": {
"signed_tx_base64": "DgAAAHNlbmRlci50ZXN0bmV0AOrmAai64SZOv9e/naX4W15pJx0GAap35wTT1T/DwcbbDwAAAAAAAAAQAAAAcmVjZWl2ZXIudGVzdG5ldNMnL7URB1cxPOu3G8jTqlEwlcasagIbKlAJlF5ywVFLAQAAAAMAAACh7czOG8LTAAAAAAAAAGQcOG03xVSFQFjoagOb4NBBqWhERnnz45LY4+52JgZhm1iQKz7qAdPByrGFDQhQ2Mfga8RlbysuQ8D8LlA6bQE=",
"wait_until": "INCLUDED_FINAL"
}
}
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=send_tx \
params:='{
"signed_tx_base64": "DgAAAHNlbmRlci50ZXN0bmV0AOrmAai64SZOv9e/naX4W15pJx0GAap35wTT1T/DwcbbDwAAAAAAAAAQAAAAcmVjZWl2ZXIudGVzdG5ldNMnL7URB1cxPOu3G8jTqlEwlcasagIbKlAJlF5ywVFLAQAAAAMAAACh7czOG8LTAAAAAAAAAGQcOG03xVSFQFjoagOb4NBBqWhERnnz45LY4+52JgZhm1iQKz7qAdPByrGFDQhQ2Mfga8RlbysuQ8D8LlA6bQE=",
"wait_until": "EXECUTED"
}'
```
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=send_tx \
params:='{
"signed_tx_base64": "DgAAAHNlbmRlci50ZXN0bmV0AOrmAai64SZOv9e/naX4W15pJx0GAap35wTT1T/DwcbbDwAAAAAAAAAQAAAAcmVjZWl2ZXIudGVzdG5ldNMnL7URB1cxPOu3G8jTqlEwlcasagIbKlAJlF5ywVFLAQAAAAMAAACh7czOG8LTAAAAAAAAAGQcOG03xVSFQFjoagOb4NBBqWhERnnz45LY4+52JgZhm1iQKz7qAdPByrGFDQhQ2Mfga8RlbysuQ8D8LlA6bQE="
}'
```
</TabItem>
</Tabs>
<details>
<summary>Example response: </summary>
<p>
```json
{
"jsonrpc": "2.0",
"result": {
"final_execution_status": "FINAL",
"status": {
"SuccessValue": ""
},
"transaction": {
"signer_id": "sender.testnet",
"public_key": "ed25519:Gowpa4kXNyTMRKgt5W7147pmcc2PxiFic8UHW9rsNvJ6",
"nonce": 13,
"receiver_id": "receiver.testnet",
"actions": [
{
"Transfer": {
"deposit": "1000000000000000000000000"
}
}
],
"signature": "ed25519:7oCBMfSHrZkT7tzPDBxxCd3tWFhTES38eks3MCZMpYPJRfPWKxJsvmwQiVBBxRLoxPTnXVaMU2jPV3MdFKZTobH",
"hash": "ASS7oYwGiem9HaNwJe6vS2kznx2CxueKDvU9BAYJRjNR"
},
"transaction_outcome": {
"proof": [],
"block_hash": "9MzuZrRPW1BGpFnZJUJg6SzCrixPpJDfjsNeUobRXsLe",
"id": "ASS7oYwGiem9HaNwJe6vS2kznx2CxueKDvU9BAYJRjNR",
"outcome": {
"logs": [],
"receipt_ids": ["BLV2q6p8DX7pVgXRtGtBkyUNrnqkNyU7iSksXG7BjVZh"],
"gas_burnt": 223182562500,
"tokens_burnt": "22318256250000000000",
"executor_id": "sender.testnet",
"status": {
"SuccessReceiptId": "BLV2q6p8DX7pVgXRtGtBkyUNrnqkNyU7iSksXG7BjVZh"
}
}
},
"receipts_outcome": [
{
"proof": [],
"block_hash": "5Hpj1PeCi32ZkNXgiD1DrW4wvW4Xtic74DJKfyJ9XL3a",
"id": "BLV2q6p8DX7pVgXRtGtBkyUNrnqkNyU7iSksXG7BjVZh",
"outcome": {
"logs": [],
"receipt_ids": ["3sawynPNP8UkeCviGqJGwiwEacfPyxDKRxsEWPpaUqtR"],
"gas_burnt": 223182562500,
"tokens_burnt": "22318256250000000000",
"executor_id": "receiver.testnet",
"status": {
"SuccessValue": ""
}
}
},
{
"proof": [],
"block_hash": "CbwEqMpPcu6KwqVpBM3Ry83k6M4H1FrJjES9kBXThcRd",
"id": "3sawynPNP8UkeCviGqJGwiwEacfPyxDKRxsEWPpaUqtR",
"outcome": {
"logs": [],
"receipt_ids": [],
"gas_burnt": 0,
"tokens_burnt": "0",
"executor_id": "sender.testnet",
"status": {
"SuccessValue": ""
}
}
}
]
},
"id": "dontcare"
}
```
</p>
</details>
#### What could go wrong? {#what-could-go-wrong-send-tx}
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `broadcast_tx_commit` method:
<table className="custom-stripe">
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr>
<td rowspan="2">HANDLER_ERROR</td>
<td>INVALID_TRANSACTION</td>
<td>An error happened during transaction execution</td>
<td>
<ul>
<li>See <code>error.cause.info</code> for details</li>
</ul>
</td>
</tr>
<tr>
<td>TIMEOUT_ERROR</td>
<td>Transaction was routed, but has not been recorded on chain in 10 seconds.</td>
<td>
<ul>
<li> Re-submit the request with the identical transaction (in NEAR Protocol unique transactions apply exactly once, so if the previously sent transaction gets applied, this request will just return the known result, otherwise, it will route the transaction to the chain once again)</li>
<li>Check that your transaction is valid</li>
<li>Check that the signer account id has enough tokens to cover the transaction fees (keep in mind that some tokens on each account are locked to cover the storage cost)</li>
</ul>
</td>
</tr>
<tr className="stripe">
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
<tr>
<td>INTERNAL_ERROR</td>
<td>INTERNAL_ERROR</td>
<td>Something went wrong with the node itself or overloaded</td>
<td>
<ul>
<li>Try again later</li>
<li>Send a request to a different node</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
---
## Transaction Status {#transaction-status}
> Queries status of a transaction by hash and returns the final transaction result.
- method: `tx`
- params:
- `tx_hash` _(see [NearBlocks Explorer](https://testnet.nearblocks.io) for a valid transaction hash)_
- `sender_account_id` _(used to determine which shard to query for transaction)_
- [Optional] `wait_until`: the required minimal execution level. Read more [here](/api/rpc/transactions#tx-status-result). The default value is `EXECUTED_OPTIMISTIC`.
A Transaction status request with `wait_until != NONE` will wait until the transaction appears on the blockchain.
If the transaction does not exist, the method will wait until the timeout is reached.
If you only need to check whether the transaction exists, use `wait_until = NONE`, it will return the response immediately.
Example:
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "tx",
"params": {
"tx_hash": "6zgh2u9DqHHiXzdy9ouTP7oGky2T4nugqzqt9wJZwNFm",
"sender_account_id": "sender.testnet",
"wait_until": "EXECUTED"
}
}
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=tx \
params:='{"tx_hash": "6zgh2u9DqHHiXzdy9ouTP7oGky2T4nugqzqt9wJZwNFm", "sender_account_id": "sender.testnet"}'
```
</TabItem>
</Tabs>
<details>
<summary>Example Result:</summary>
<p>
```json
{
"jsonrpc": "2.0",
"result": {
"final_execution_status": "FINAL",
"status": {
"SuccessValue": ""
},
"transaction": {
"signer_id": "sender.testnet",
"public_key": "ed25519:Gowpa4kXNyTMRKgt5W7147pmcc2PxiFic8UHW9rsNvJ6",
"nonce": 15,
"receiver_id": "receiver.testnet",
"actions": [
{
"Transfer": {
"deposit": "1000000000000000000000000"
}
}
],
"signature": "ed25519:3168QMdTpcwHvM1dmMYBc8hg9J3Wn8n7MWBSE9WrEpns6P5CaY87RM6k4uzyBkQuML38CZhU18HzmQEevPG1zCvk",
"hash": "6zgh2u9DqHHiXzdy9ouTP7oGky2T4nugqzqt9wJZwNFm"
},
"transaction_outcome": {
"proof": [
{
"hash": "F7mL76CMdfbdZ3xCehVGNh1fCyaR37gr3MeGX3EZkiVf",
"direction": "Right"
}
],
"block_hash": "ADTMLVtkhsvzUxuf6m87Pt1dnF5vi1yY7ftxmNpFx7y",
"id": "6zgh2u9DqHHiXzdy9ouTP7oGky2T4nugqzqt9wJZwNFm",
"outcome": {
"logs": [],
"receipt_ids": ["3dMfwczW5GQqXbD9GMTnmf8jy5uACxG6FC5dWxm3KcXT"],
"gas_burnt": 223182562500,
"tokens_burnt": "22318256250000000000",
"executor_id": "sender.testnet",
"status": {
"SuccessReceiptId": "3dMfwczW5GQqXbD9GMTnmf8jy5uACxG6FC5dWxm3KcXT"
}
}
},
"receipts_outcome": [
{
"proof": [
{
"hash": "6h95oEd7ih62KXfyPT4zsZYont4qy9sWEXc5VQVDhqtG",
"direction": "Right"
},
{
"hash": "6DnibgZk1T669ZprcehUy1GpCSPw1kjzXRGu69nSaUNn",
"direction": "Right"
}
],
"block_hash": "GgFTVr33r4MrpAiHc9mr8TZqLnpZAX1BaZTNvhBnciy2",
"id": "3dMfwczW5GQqXbD9GMTnmf8jy5uACxG6FC5dWxm3KcXT",
"outcome": {
"logs": [],
"receipt_ids": ["46KYgN8ddxs4Qy8C7BDQH49XUfcYZsaQmAvdU1nfcL9V"],
"gas_burnt": 223182562500,
"tokens_burnt": "22318256250000000000",
"executor_id": "receiver.testnet",
"status": {
"SuccessValue": ""
}
}
},
{
"proof": [
{
"hash": "CD9Y7Bw3MSFgaPZzpc1yP51ajhGDCAsR61qXcMNcRoHf",
"direction": "Left"
}
],
"block_hash": "EGAgKuW6Bd6QKYSaxAkx2pPGmnjrjAcq4UpoUiqMXvPH",
"id": "46KYgN8ddxs4Qy8C7BDQH49XUfcYZsaQmAvdU1nfcL9V",
"outcome": {
"logs": [],
"receipt_ids": [],
"gas_burnt": 0,
"tokens_burnt": "0",
"executor_id": "sender.testnet",
"status": {
"SuccessValue": ""
}
}
}
]
},
"id": "dontcare"
}
```
</p>
</details>
#### What could go wrong? {#what-could-go-wrong-2}
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `tx` method:
<table className="custom-stripe">
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr>
<td rowspan="3">HANDLER_ERROR</td>
<td>INVALID_TRANSACTION</td>
<td>An error happened during transaction execution</td>
<td>
<ul>
<li>See <code>error.cause.info</code> for details</li>
</ul>
</td>
</tr>
<tr>
<td>UNKNOWN_TRANSACTION</td>
<td>The requested transaction is not available on the node since it might have not been recorded on the chain yet or has been garbage-collected</td>
<td>
<ul>
<li>Try again later</li>
<li>If the transaction had been submitted more than 5 epochs ago, try to send your request to <a href="https://near-nodes.io/intro/node-types#archival-node">an archival node</a></li>
<li>Check the transaction hash</li>
</ul>
</td>
</tr>
<tr>
<td>TIMEOUT_ERROR</td>
<td>It was unable to wait for the transaction status for reasonable time</td>
<td>
<ul>
<li>Send a request to a different node</li>
<li>Try again later</li>
</ul>
</td>
</tr>
<tr className="stripe">
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
<tr>
<td>INTERNAL_ERROR</td>
<td>INTERNAL_ERROR</td>
<td>Something went wrong with the node itself or overloaded</td>
<td>
<ul>
<li>Try again later</li>
<li>Send a request to a different node</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
---
## Transaction Status with Receipts {#transaction-status-with-receipts}
> Queries status of a transaction by hash, returning the final transaction result _and_ details of all receipts.
- method: `EXPERIMENTAL_tx_status`
- params:
- `tx_hash` _(see [NearBlocks Explorer](https://testnet.nearblocks.io) for a valid transaction hash)_
- `sender_account_id` _(used to determine which shard to query for transaction)_
- [Optional] `wait_until`: the required minimal execution level. Read more [here](/api/rpc/transactions#tx-status-result). The default value is `EXECUTED_OPTIMISTIC`.
A Transaction status request with `wait_until != NONE` will wait until the transaction appears on the blockchain.
If the transaction does not exist, the method will wait until the timeout is reached.
If you only need to check whether the transaction exists, use `wait_until = NONE`, it will return the response immediately.
Example:
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "EXPERIMENTAL_tx_status",
"params": {
"tx_hash": "HEgnVQZfs9uJzrqTob4g2Xmebqodq9waZvApSkrbcAhd",
"sender_account_id": "bowen",
"wait_until": "EXECUTED"
}
}
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 method=EXPERIMENTAL_tx_status params:='{"tx_hash": "HEgnVQZfs9uJzrqTob4g2Xmebqodq9waZvApSkrbcAhd", "sender_account_id": "bowen"}' id=dontcare
```
</TabItem>
</Tabs>
<details>
<summary>Example response:</summary>
<p>
```json
{
"id": "123",
"jsonrpc": "2.0",
"result": {
"final_execution_status": "FINAL",
"receipts": [
{
"predecessor_id": "bowen",
"receipt": {
"Action": {
"actions": [
{
"FunctionCall": {
"args": "eyJhbW91bnQiOiIxMDAwIiwicmVjZWl2ZXJfaWQiOiJib3dlbiJ9",
"deposit": "0",
"gas": 100000000000000,
"method_name": "transfer"
}
}
],
"gas_price": "186029458",
"input_data_ids": [],
"output_data_receivers": [],
"signer_id": "bowen",
"signer_public_key": "ed25519:2f9Zv5kuyuPM5DCyEP5pSqg58NQ8Ct9uSRerZXnCS9fK"
}
},
"receipt_id": "FXMVxdhSUZaZftbmPJWaoqhEB9GrKB2oqg9Wgvuyvom8",
"receiver_id": "evgeny.lockup.m0"
},
{
"predecessor_id": "evgeny.lockup.m0",
"receipt": {
"Action": {
"actions": [
{
"Transfer": {
"deposit": "1000"
}
}
],
"gas_price": "186029458",
"input_data_ids": [],
"output_data_receivers": [],
"signer_id": "bowen",
"signer_public_key": "ed25519:2f9Zv5kuyuPM5DCyEP5pSqg58NQ8Ct9uSRerZXnCS9fK"
}
},
"receipt_id": "3Ad7pUygUegMUWUb1rEazfjnTaHfptXCABqKQ6WNq6Wa",
"receiver_id": "bowen"
},
{
"predecessor_id": "system",
"receipt": {
"Action": {
"actions": [
{
"Transfer": {
"deposit": "19200274886926125000"
}
}
],
"gas_price": "0",
"input_data_ids": [],
"output_data_receivers": [],
"signer_id": "bowen",
"signer_public_key": "ed25519:2f9Zv5kuyuPM5DCyEP5pSqg58NQ8Ct9uSRerZXnCS9fK"
}
},
"receipt_id": "5DdQg9pfoJMX1q6bvhsjyyRihzA3sb9Uq5K1J7vK43Ze",
"receiver_id": "bowen"
},
{
"predecessor_id": "system",
"receipt": {
"Action": {
"actions": [
{
"Transfer": {
"deposit": "18663792669276228632284"
}
}
],
"gas_price": "0",
"input_data_ids": [],
"output_data_receivers": [],
"signer_id": "bowen",
"signer_public_key": "ed25519:2f9Zv5kuyuPM5DCyEP5pSqg58NQ8Ct9uSRerZXnCS9fK"
}
},
"receipt_id": "FDp8ovTf5uJYDFemW5op6ebjCT2n4CPExHYie3S1h4qp",
"receiver_id": "bowen"
}
],
"receipts_outcome": [
{
"block_hash": "HuqYrYsC7h2VERFMgFkqaNqSiFuTH9CA3uJr3BkyNxhF",
"id": "FXMVxdhSUZaZftbmPJWaoqhEB9GrKB2oqg9Wgvuyvom8",
"outcome": {
"executor_id": "evgeny.lockup.m0",
"gas_burnt": 3493189769144,
"logs": ["Transferring 1000 to account @bowen"],
"receipt_ids": [
"3Ad7pUygUegMUWUb1rEazfjnTaHfptXCABqKQ6WNq6Wa",
"FDp8ovTf5uJYDFemW5op6ebjCT2n4CPExHYie3S1h4qp"
],
"status": {
"SuccessReceiptId": "3Ad7pUygUegMUWUb1rEazfjnTaHfptXCABqKQ6WNq6Wa"
},
"tokens_burnt": "349318976914400000000"
},
"proof": [
{
"direction": "Right",
"hash": "5WwHEszBcpfrHnt2VTvVDVnEEACNq5EpQdjz1aW9gTAa"
}
]
},
{
"block_hash": "DJ6oK5FtPPSwksS6pKdEjFvHWAaSVocnVNLoyi8aYk1k",
"id": "3Ad7pUygUegMUWUb1rEazfjnTaHfptXCABqKQ6WNq6Wa",
"outcome": {
"executor_id": "bowen",
"gas_burnt": 223182562500,
"logs": [],
"receipt_ids": ["5DdQg9pfoJMX1q6bvhsjyyRihzA3sb9Uq5K1J7vK43Ze"],
"status": {
"SuccessValue": ""
},
"tokens_burnt": "22318256250000000000"
},
"proof": [
{
"direction": "Right",
"hash": "CXSXmKpDU8R3UUrBAsffWMeGfKanKqEHCQrHeZkR3RKT"
},
{
"direction": "Right",
"hash": "2dNo7A1VHKBmMA86m1k3Z9DVXwWgQJGkKGRg8wUR3co9"
}
]
},
{
"block_hash": "9cjUoqAksMbs7ZJ4CXiuwm8vppz9QctTwGmgwZ5mDmUA",
"id": "5DdQg9pfoJMX1q6bvhsjyyRihzA3sb9Uq5K1J7vK43Ze",
"outcome": {
"executor_id": "bowen",
"gas_burnt": 0,
"logs": [],
"receipt_ids": [],
"status": {
"SuccessValue": ""
},
"tokens_burnt": "0"
},
"proof": []
},
{
"block_hash": "DJ6oK5FtPPSwksS6pKdEjFvHWAaSVocnVNLoyi8aYk1k",
"id": "FDp8ovTf5uJYDFemW5op6ebjCT2n4CPExHYie3S1h4qp",
"outcome": {
"executor_id": "bowen",
"gas_burnt": 0,
"logs": [],
"receipt_ids": [],
"status": {
"SuccessValue": ""
},
"tokens_burnt": "0"
},
"proof": [
{
"direction": "Left",
"hash": "A2Ry6NCeuK8WhRCWc41hy6uddadc5nLJ1NBX5wVYo3Yb"
},
{
"direction": "Right",
"hash": "2dNo7A1VHKBmMA86m1k3Z9DVXwWgQJGkKGRg8wUR3co9"
}
]
}
],
"status": {
"SuccessValue": ""
},
"transaction": {
"actions": [
{
"FunctionCall": {
"args": "eyJhbW91bnQiOiIxMDAwIiwicmVjZWl2ZXJfaWQiOiJib3dlbiJ9",
"deposit": "0",
"gas": 100000000000000,
"method_name": "transfer"
}
}
],
"hash": "HEgnVQZfs9uJzrqTob4g2Xmebqodq9waZvApSkrbcAhd",
"nonce": 77,
"public_key": "ed25519:2f9Zv5kuyuPM5DCyEP5pSqg58NQ8Ct9uSRerZXnCS9fK",
"receiver_id": "evgeny.lockup.m0",
"signature": "ed25519:5v1hJuw5RppKGezJHBFU6z3hwmmdferETud9rUbwxVf6xSBAWyiod93Lezaq4Zdcp4zbukDusQY9PjhV47JVCgBx",
"signer_id": "bowen"
},
"transaction_outcome": {
"block_hash": "9RX2pefXKw8M4EYjLznDF3AMvbkf9asAjN8ACK7gxKsa",
"id": "HEgnVQZfs9uJzrqTob4g2Xmebqodq9waZvApSkrbcAhd",
"outcome": {
"executor_id": "bowen",
"gas_burnt": 2428026088898,
"logs": [],
"receipt_ids": ["FXMVxdhSUZaZftbmPJWaoqhEB9GrKB2oqg9Wgvuyvom8"],
"status": {
"SuccessReceiptId": "FXMVxdhSUZaZftbmPJWaoqhEB9GrKB2oqg9Wgvuyvom8"
},
"tokens_burnt": "242802608889800000000"
},
"proof": [
{
"direction": "Right",
"hash": "DXf4XVmAF5jnjZhcxi1CYxGPuuQrcAmayq9X5inSAYvJ"
}
]
}
}
}
```
</p>
</details>
#### What could go wrong? {#what-could-go-wrong-3}
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `EXPERIMENTAL_tx_status` method:
<table className="custom-stripe">
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr>
<td rowspan="3">HANDLER_ERROR</td>
<td>INVALID_TRANSACTION</td>
<td>An error happened during transaction execution</td>
<td>
<ul>
<li>See <code>error.cause.info</code> for details</li>
</ul>
</td>
</tr>
<tr>
<td>UNKNOWN_TRANSACTION</td>
<td>The requested transaction is not available on the node since it might have not been recorded on the chain yet or has been garbage-collected</td>
<td>
<ul>
<li>Try again later</li>
<li>If the transaction had been submitted more than 5 epochs ago, try to send your request to <a href="https://near-nodes.io/intro/node-types#archival-node">an archival node</a></li>
<li>Check the transaction hash</li>
</ul>
</td>
</tr>
<tr>
<td>TIMEOUT_ERROR</td>
<td>It was unable to wait for the transaction status for reasonable time</td>
<td>
<ul>
<li>Send a request to a different node</li>
<li>Try again later</li>
</ul>
</td>
</tr>
<tr className="stripe">
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
<tr>
<td>INTERNAL_ERROR</td>
<td>INTERNAL_ERROR</td>
<td>Something went wrong with the node itself or overloaded</td>
<td>
<ul>
<li>Try again later</li>
<li>Send a request to a different node</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
---
### Receipt by ID {#receipt-by-id}
> Fetches a receipt by it's ID (as is, without a status or execution outcome)
- method: `EXPERIMENTAL_receipt`
- params:
- `receipt_id` _(see [NearBlocks Explorer](https://testnet.nearblocks.io) for a valid receipt id)_
Example:
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "EXPERIMENTAL_receipt",
"params": { "receipt_id": "2EbembRPJhREPtmHCrGv3Xtdm3xoc5BMVYHm3b2kjvMY" }
}
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 method=EXPERIMENTAL_receipt params:='{"receipt_id": "2EbembRPJhREPtmHCrGv3Xtdm3xoc5BMVYHm3b2kjvMY"}' id=dontcare
```
</TabItem>
</Tabs>
<details>
<summary>Example response:</summary>
<p>
```json
{
"id": "dontcare",
"jsonrpc": "2.0",
"result": {
"predecessor_id": "bohdan.testnet",
"receipt": {
"Action": {
"actions": [
{
"Transfer": {
"deposit": "1000000000000000000000000"
}
}
],
"gas_price": "103000000",
"input_data_ids": [],
"output_data_receivers": [],
"signer_id": "bohdan.testnet",
"signer_public_key": "ed25519:DhC7rPNTBwWJtmVXs1U1SqJztkn9AWbj6jCmQtkrg3TA"
}
},
"receipt_id": "2EbembRPJhREPtmHCrGv3Xtdm3xoc5BMVYHm3b2kjvMY",
"receiver_id": "frol.testnet"
}
}
```
</p>
</details>
#### What could go wrong? {#what-could-go-wrong-4}
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `EXPERIMENTAL_receipt` method:
<table>
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr>
<td>HANDLER_ERROR</td>
<td>UNKNOWN_RECEIPT</td>
<td>The receipt with the given <code>receipt_id</code> was never observed on the node</td>
<td>
<ul>
<li>Check the provided <code>receipt_id</code> is correct</li>
<li>Send a request on a different node</li>
</ul>
</td>
</tr>
<tr>
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
<tr>
<td>INTERNAL_ERROR</td>
<td>INTERNAL_ERROR</td>
<td>Something went wrong with the node itself or overloaded</td>
<td>
<ul>
<li>Try again later</li>
<li>Send a request to a different node</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
## Transaction Execution Levels {#tx-status-result}
All the methods listed above have `wait_until` request parameter, and `final_execution_status` response value.
They correspond to the same enum `TxExecutionStatus`.
See the detailed explanation for all the options:
```rust
#[serde(rename_all = "SCREAMING_SNAKE_CASE")]
pub enum TxExecutionStatus {
/// Transaction is waiting to be included into the block
None,
/// Transaction is included into the block. The block may be not finalized yet
Included,
/// Transaction is included into the block +
/// All non-refund transaction receipts finished their execution.
/// The corresponding blocks for tx and each receipt may be not finalized yet
#[default]
ExecutedOptimistic,
/// Transaction is included into finalized block
IncludedFinal,
/// Transaction is included into finalized block +
/// All non-refund transaction receipts finished their execution.
/// The corresponding blocks for each receipt may be not finalized yet
Executed,
/// Transaction is included into finalized block +
/// Execution of all transaction receipts is finalized, including refund receipts
Final,
}
```
---
# Deprecated methods {#deprecated}
## [deprecated] Send transaction (async) {#send-transaction-async}
> Consider using [`send_tx`](/api/rpc/transactions#send-tx) instead
> Sends a transaction and immediately returns transaction hash.
- method: `broadcast_tx_async`
- params: [SignedTransaction encoded in base64]
Example:
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "broadcast_tx_async",
"params": [
"DgAAAHNlbmRlci50ZXN0bmV0AOrmAai64SZOv9e/naX4W15pJx0GAap35wTT1T/DwcbbDwAAAAAAAAAQAAAAcmVjZWl2ZXIudGVzdG5ldNMnL7URB1cxPOu3G8jTqlEwlcasagIbKlAJlF5ywVFLAQAAAAMAAACh7czOG8LTAAAAAAAAAGQcOG03xVSFQFjoagOb4NBBqWhERnnz45LY4+52JgZhm1iQKz7qAdPByrGFDQhQ2Mfga8RlbysuQ8D8LlA6bQE="
]
}
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=broadcast_tx_async \
params:='[
"DgAAAHNlbmRlci50ZXN0bmV0AOrmAai64SZOv9e/naX4W15pJx0GAap35wTT1T/DwcbbDwAAAAAAAAAQAAAAcmVjZWl2ZXIudGVzdG5ldNMnL7URB1cxPOu3G8jTqlEwlcasagIbKlAJlF5ywVFLAQAAAAMAAACh7czOG8LTAAAAAAAAAGQcOG03xVSFQFjoagOb4NBBqWhERnnz45LY4+52JgZhm1iQKz7qAdPByrGFDQhQ2Mfga8RlbysuQ8D8LlA6bQE="
]'
```
</TabItem>
</Tabs>
Example response:
```json
{
"jsonrpc": "2.0",
"result": "6zgh2u9DqHHiXzdy9ouTP7oGky2T4nugqzqt9wJZwNFm",
"id": "dontcare"
}
```
Final transaction results can be queried using [Transaction Status](#transaction-status)
or [NearBlocks Explorer](https://testnet.nearblocks.io/) using the above `result` hash returning a result similar to the example below.

#### What could go wrong? {#what-could-go-wrong}
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `broadcast_tx_async` method:
<table class="custom-stripe">
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr class="stripe">
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
---
## [deprecated] Send transaction (await) {#send-transaction-await}
> Consider using [`send_tx`](/api/rpc/transactions#send-tx) instead
> Sends a transaction and waits until transaction is fully complete. _(Has a 10 second timeout)_
- method: `broadcast_tx_commit`
- params: `[SignedTransaction encoded in base64]`
Example:
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "broadcast_tx_commit",
"params": [
"DgAAAHNlbmRlci50ZXN0bmV0AOrmAai64SZOv9e/naX4W15pJx0GAap35wTT1T/DwcbbDQAAAAAAAAAQAAAAcmVjZWl2ZXIudGVzdG5ldIODI4YfV/QS++blXpQYT+bOsRblTRW4f547y/LkvMQ9AQAAAAMAAACh7czOG8LTAAAAAAAAAAXcaTJzu9GviPT7AD4mNJGY79jxTrjFLoyPBiLGHgBi8JK1AnhK8QknJ1ourxlvOYJA2xEZE8UR24THmSJcLQw="
]
}
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=broadcast_tx_commit \
params:='[
"DwAAAG5lYXJrYXQudGVzdG5ldABuTi5L1rwnlb35hc9tn5WELkxfiGfGh1Q5aeGNQDejo0QAAAAAAAAAEAAAAGpvc2hmb3JkLnRlc3RuZXSiWAc6W9KlqXS5fK+vjFRDV5pAxHRKU0srKX/cmdRTBgEAAAADAAAAoe3MzhvC0wAAAAAAAAB9rOE9zc5zQYLL1j6VTh3I4fQbERs6I07gJfrAC6jo8DB4HolR9Xps3v4qrZxkgZjwv6wB0QOROM4UEbeOaBoB"
]'
```
</TabItem>
</Tabs>
<details>
<summary>Example response: </summary>
<p>
```json
{
"jsonrpc": "2.0",
"result": {
"final_execution_status": "FINAL",
"status": {
"SuccessValue": ""
},
"transaction": {
"signer_id": "sender.testnet",
"public_key": "ed25519:Gowpa4kXNyTMRKgt5W7147pmcc2PxiFic8UHW9rsNvJ6",
"nonce": 13,
"receiver_id": "receiver.testnet",
"actions": [
{
"Transfer": {
"deposit": "1000000000000000000000000"
}
}
],
"signature": "ed25519:7oCBMfSHrZkT7tzPDBxxCd3tWFhTES38eks3MCZMpYPJRfPWKxJsvmwQiVBBxRLoxPTnXVaMU2jPV3MdFKZTobH",
"hash": "ASS7oYwGiem9HaNwJe6vS2kznx2CxueKDvU9BAYJRjNR"
},
"transaction_outcome": {
"proof": [],
"block_hash": "9MzuZrRPW1BGpFnZJUJg6SzCrixPpJDfjsNeUobRXsLe",
"id": "ASS7oYwGiem9HaNwJe6vS2kznx2CxueKDvU9BAYJRjNR",
"outcome": {
"logs": [],
"receipt_ids": ["BLV2q6p8DX7pVgXRtGtBkyUNrnqkNyU7iSksXG7BjVZh"],
"gas_burnt": 223182562500,
"tokens_burnt": "22318256250000000000",
"executor_id": "sender.testnet",
"status": {
"SuccessReceiptId": "BLV2q6p8DX7pVgXRtGtBkyUNrnqkNyU7iSksXG7BjVZh"
}
}
},
"receipts_outcome": [
{
"proof": [],
"block_hash": "5Hpj1PeCi32ZkNXgiD1DrW4wvW4Xtic74DJKfyJ9XL3a",
"id": "BLV2q6p8DX7pVgXRtGtBkyUNrnqkNyU7iSksXG7BjVZh",
"outcome": {
"logs": [],
"receipt_ids": ["3sawynPNP8UkeCviGqJGwiwEacfPyxDKRxsEWPpaUqtR"],
"gas_burnt": 223182562500,
"tokens_burnt": "22318256250000000000",
"executor_id": "receiver.testnet",
"status": {
"SuccessValue": ""
}
}
},
{
"proof": [],
"block_hash": "CbwEqMpPcu6KwqVpBM3Ry83k6M4H1FrJjES9kBXThcRd",
"id": "3sawynPNP8UkeCviGqJGwiwEacfPyxDKRxsEWPpaUqtR",
"outcome": {
"logs": [],
"receipt_ids": [],
"gas_burnt": 0,
"tokens_burnt": "0",
"executor_id": "sender.testnet",
"status": {
"SuccessValue": ""
}
}
}
]
},
"id": "dontcare"
}
```
</p>
</details>
#### What could go wrong? {#what-could-go-wrong-1}
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `broadcast_tx_commit` method:
<table class="custom-stripe">
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr>
<td rowspan="2">HANDLER_ERROR</td>
<td>INVALID_TRANSACTION</td>
<td>An error happened during transaction execution</td>
<td>
<ul>
<li>See <code>error.cause.info</code> for details</li>
</ul>
</td>
</tr>
<tr>
<td>TIMEOUT_ERROR</td>
<td>Transaction was routed, but has not been recorded on chain in 10 seconds.</td>
<td>
<ul>
<li> Re-submit the request with the identical transaction (in NEAR Protocol unique transactions apply exactly once, so if the previously sent transaction gets applied, this request will just return the known result, otherwise, it will route the transaction to the chain once again)</li>
<li>Check that your transaction is valid</li>
<li>Check that the signer account id has enough tokens to cover the transaction fees (keep in mind that some tokens on each account are locked to cover the storage cost)</li>
</ul>
</td>
</tr>
<tr class="stripe">
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
<tr>
<td>INTERNAL_ERROR</td>
<td>INTERNAL_ERROR</td>
<td>Something went wrong with the node itself or overloaded</td>
<td>
<ul>
<li>Try again later</li>
<li>Send a request to a different node</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
---
|
NEAR Launches Balkans Regional Hub to Incubate Web3 Talent and Projects
COMMUNITY
June 1, 2022
Fostering Regional Hubs around the world is an important part of NEAR Foundation’s mission. Fresh off the Kenya Regional Hub launch, the foundation has reached another milestone in the Regional Hubs strategy.
This week, NEAR is excited to announce the launch of the NEAR Balkans Regional Hub, which will accelerate onboarding of Web3 talents and blockchain projects in the Balkans. The Balkans Regional Hub is only the latest, though certainly not the last, node Borderless Tech Associationin the NEAR Foundation’s ongoing efforts to globalize and democratize the Web3 industry.
Web3 growth and talent in the Balkans
NEAR Balkans Regional Hub gathering in Belgrade, Serbia, May 2022
The Balkans is home to an incredibly talented and tech-savvy community. NEAR Balkans will tap into this deep pool of talent and creativity, accelerating the development of new Web3 projects and skills throughout the region.
Beyond the region’s deep developer talent pool, the Balkans is also home to a growing community that includes startups and established businesses. Even before the hub’s launch, the Balkans had a number of Web3 success stories, attracting investor and global VC attention.
Taken together, the region’s talent and startups made the Balkans an ideal node in NEAR’s Regional Hub network.
NEAR Balkan’s mission
Established in partnership with the , NEAR Balkans provides the local community with tools and support to begin building on the NEAR protocol. The hub will be a resource for developers and entrepreneurs looking to educate themselves about the technology, and to expand the NEAR ecosystem.
NEAR Balkans will also serve as a funnel for NEAR Foundation Grants. These grants will be made available to developers, entrepreneurs, and Web2 businesses with promising and innovative ideas for Web3 apps.
NEAR Balkans attendees in Belgrade, Serbia, May 2022
The Balkans hub will also guide founders, developers, and community members throughout their journey. Beyond funding and education, NEAR Balkans will facilitate partnerships, mentoring, and incubation for both individuals and teams. The hub will also focus on launching new products from its innovation lab. The aim is to inspire the region’s entrepreneurs, and showcase why building in a more decentralized and democratized way is the future.
The Hub will drive activities and cover the following countries: Bosnia & Herzegovina, Bulgaria, Croatia, Montenegro, North Macedonia, Serbia and Slovenia.
The NEAR Balkans team
The NEAR Balkans Hub features a team of more than 15 Web3 experts and blockchain enthusiasts. Team members come from a variety of backgrounds, including business, education, product labs and more.
Collectively, the NEAR Balkans team members will leverage their Web3 experience in managing projects in the Balkan region. These efforts are crucial in driving the NEAR ecosystem’s growth, not only in the Balkans but also globally.
NEAR Balkans attendees in Zagreb, Croatia, May 2022
“We are dedicated to bringing the best of education and opportunities for companies and individuals interested in Web3 through strong local support across our Balkan region, which is full of creative, technical and business talents,” says Ida Pandur, General Manager of NEAR Balkans. We are looking forward to forming strong partnerships with teams, businesses and universities to bring the mission to execution.”
An inspiring launch in the Balkans
Since coalescing six months ago, NEAR Balkans is officially launching on a high note. From Zagreb, Croatia to Belgrade, Serbia and Sofia, Bulgaria, the hub is lively base of developers, entrepreneurs, and students participating in a number of activities, from meetups to hackathons and internships.
The hub has also launched several products on NEAR from its innovation lab, focusing on Web3 arenas such DeFi and NFTs. One such project is Electrocoin, the company behind Bitcoin Mjenjacnica, one of the biggest crypto exchanges in the region. Electrocoin enables customers to transfer money from crypto to fiat and vice versa. The app will also allow users to pay their bills and invoices.
NEAR Balkans attendees in Sofia, Bulgaria, May 2022
Another project, CircuitMess, is building innovative DIY STEM toys for kids, and are currently at work on NFT integration. CircuitMess wants to equip children with the skills to build on Web3 today.
The NEAR Balkans hub includes regional ambassadors, who attend meetups and share valuable information and contacts to anyone interested in learning or building on NEAR. There are tentative plans to hold 3 to 5 meetups a month across different Balkans cities, as well as two live hackathons in the coming months.
Much like the NEAR Ukraine Regional Hub, NEAR Balkans is able to reach a large pool of developers. The hub is particularly focused on leveraging this talent pool for its Web3 education efforts, including workshops and collaborations with regional universities. It is also funneling students from a variety of backgrounds into the NEAR Certified Developer program.
NEAR Balkans is only the beginning of what will be a long and prosperous journey of Web3 innovation in the region. |
Thanksgiving with NEAR | November 29th, 2019
COMMUNITY
November 29, 2019
If you are celebrating Thanksgiving, we hope that you have an amazing time. Our team has taken the holiday off but there is always time for a community update. So here it is – Enjoy!
In the spirit of Thanksgiving, we want to give a shout out to our community i.e. to You! We are building NEAR with a large ecosystem of developers, users, and supporters. Without your feedback, support and contribution, we would not have been able to move at this pace.
Our warriors are still going strong on Stake Wars. Fun fact, someone launched a phishing attack (stay sharp and don’t get fooled). You can read more on last week’s retrospective here. Note that we are publishing a retrospective every week on our blog.
Consistency is key! We are working hard on providing more educational material on NEAR Protocol. Continuing, the fourth NEAR Lunch and Learn Episode is out and focuses on Nightshade. Alex covers block production, approvals, separation of transactions into chunks, data availability, and finality.
Happy Thanksgiving! Photo by Randy Fath on Unsplash
COMMUNITY UPDATE
We are heavily working on community programs and redefining the structure and goal of existing ones. Stay tuned for further updates.
Anais Urlichs (i.e. me) showed off some of the developer tools available on NEAR and gave a sneak peek into some of the new documentation at LederZ’s developer meetup in Berlin.
UPCOMING EVENTS
Next up, our second Hack Night! We had a lot of fun welcoming community members in our office in San Francisco. This Wednesday is the second edition. Not only are we able to provide 1-to-1 support and answer dev questions but the feedback also allows us to improve NEAR developer tools and the documentation.
If you are unable to come, we would still love to hear from you! Your feedback will help us shape the developer experience on NEAR.
Anais showing off the Docs
WRITING AND CONTENT
If you want to learn more on how to get started building smart contracts on NEAR, Colin Eberhardt shared his experience in his post “WebAssembly on the Blockchain and JavaScript Smart Contracts”.
The fourth episode of our “Lunch and Learn” series is out. This time Alex is focusing on Nightshade, NEAR’s consensus design.
Curious how Stake Wars is going and how you can get involved? Here is last week’s retrospective; also available in Chinese, translated by one of our amazing ambassadors. For further updates, join our Discord.
Answer to “What gets you excited about the crypto space?” by Sasha
ENGINEERING UPDATES
178 PRs across 25 repos by 25 authors. Featured repos:nearcore,nearlib,near-shell,near-wallet,near-bindgen, assemblyscript-json, nomicon, near-fiddle-api, near-runtime-ts, near-contract-helper, borsh, stakewars and near-explorer;
Published v0.4.7 of nearcore
VRF implementation
Runtime stability improvement
Nightly test infrastructure
Making the Finality Gadget respect weights in nearcore
Release binary to gcloud in nearcore
Version 0.17.2 of nearlib
Switch rewards to 30% for function call only in nearcore
Allow genesis tool to specify shards to track in nearcore
Allow having conditional compilation and other method level attributes in near-bindgen
Release of version 0.14.2 of near-shell
We now have a search box in near-explorer
Expose locked balance to smart contracts in near-bindgen
Dashboard transaction history in near-wallet
We’ve hit the 400 stars mark on GitHub!
https://upscri.be/633436/
|
---
id: types
title: SDK Types
hide_table_of_contents: true
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
import {CodeTabs, Language, Github} from "@site/src/components/codetabs"
import CodeBlock from '@theme/CodeBlock'
import {ExplainCode, Block, File} from '@site/src/components/CodeExplainer/code-explainer';
Lets discuss which types smart contracts use to input and output data, as well as how such data is stored and handled in the contract's code.
<ExplainCode languages={["js", "rust"]}>
<Block highlights={{"js":"5,8,13"}} fname="hello-near">
### Native Types
Smart contracts can receive, store and return data using JS native types:
- `string`
- `number`
- `boolean`
- `Array`
- `Map`
- `Object`
- `BigInt`
</Block>
<Block highlights={{"rust":"6,13,22,27"}} fname="hello-near">
### Native Types
Smart contracts can receive, store and return data using the following Rust types:
- `string`
- `i8-i32/u8-u32`
- **`u64/128`**: It is preferable to use SDK types `U64` and `U128`
- `bool`
- `HashMap`
- `Vector`
</Block>
<Block highlights={{"rust": "1,15,22,64"}} fname="auction">
#### `U64/U128`
Smart contracts can store `u64` and `u128`, but these types need to be converted to `string` for input/output
To simplify development, the SDK provides the `U64` and `U128` types which are automatically casted to `u64/u128` when stored, and to `string` when used as input/output
</Block>
<Block highlights={{"js":"3-6", "rust": "6-9"}} fname="auction">
### Complex Objects
Smart contracts can store and return complex objects
**Note:** Objects will always be received and returned as JSON
</Block>
<Block highlights={{"rust": "4"}} fname="auction">
#### Serializers
Objects that will be used as input or output need to be serializable to JSON, add the `#[near(serializer=json)]` macro
Objects that will be stored in the contract's state need to be serializable to Borsh, add the `#[near(serializer=borsh)]` macro
</Block>
<Block highlights={{"js": "5,11,47"}} fname="auction">
### Handling Tokens
`$NEAR` tokens are represented using `BigInt` in JS, and they are always represented in `yoctonear`
**Note:** 1 NEAR = 10^24 yoctoNEAR
</Block>
<Block highlights={{"rust": ""}} fname="auction">
### Handling Tokens
`$NEAR` tokens are handled through the `NearToken` struct, which exposes methods to represent the value in `yoctonear`, `milinear` and `near`
**Note:** 1 NEAR = 10^24 yoctonear
</Block>
<Block highlights={{"js": "4", "rust": "7"}} fname="auction">
### Account
The SDK exposes a special type to handle NEAR Accounts, which automatically checks if the account address is valid
</Block>
<File
language="js"
fname="hello-near"
url="https://github.com/near-examples/hello-near-examples/blob/main/contract-ts/src/contract.ts"
start="2"
end="18"
/>
<File
language="rust"
fname="hello-near"
url="https://github.com/near-examples/hello-near-examples/blob/main/contract-rs/src/lib.rs"
start="2"
end="32"
/>
<File
language="js"
fname="auction"
url="https://github.com/near-examples/auction-examples/blob/main/contract-ts/src/contract.ts"
start="2"
end="51"
/>
<File
language="rust"
fname="auction"
url="https://github.com/near-examples/auction-examples/blob/main/contract-rs/src/lib.rs"
start="2"
end="68"
/>
</ExplainCode> |
The authoritative guide to Blockchain Sharding, part 1
DEVELOPERS
December 5, 2018
This blog post is the first in the series of two on Blockchain Sharding. After reading this blog post you will know why Sharding is the path to the future-proof blockchain protocols, how Sharding is being built today, what challenges all the sharded protocols face, and how such challenges can be addressed. The second post covers more advanced topics such as data availability, data validity and corrupting shards.
It is well-known that Ethereum, the most used general purpose blockchain at the time of this writing, can only process less than 20 transactions per second on the main chain. This limitation, coupled with the popularity of the network, leads to high gas prices (the cost of executing a transaction on the network) and long confirmation times; despite the fact that at the time of this writing a new block is produced approximately every 10–20 seconds the average time it actually takes for a transaction to be added to the blockchain is 1.2 minutes, according to ETH Gas Station. Low throughput, high prices, and high latency all make Ethereum not suitable to run services that need to scale with adoption.
What is the primary reason for Ethereum’s low throughput? The reason is that every node in the network needs to process every single transaction. Developers have proposed many solutions to address the issue of throughput on the protocol level. These solutions can be mostly separated into those that delegate all the computation to a small set of powerful nodes, and those that have each node in the network only do a subset of the total amount of work. An extreme case of the former approach is Thunder that has one single node processing all the transactions and claims to achieve 1200 tx/sec, a 100x improvement over Ethereum (I do not, however, endorse Thunder, or attest to the validity of their claims). Algorand, SpaceMesh, Solana all fit into the former category, building various improvements in the consensus and the structure of the blockchain itself to run significantly more transactions, but still bounded by what a single (albeit very powerful) machine can process.
The latter approach, in which the work is split among all the participating nodes, is called sharding. This is how Ethereum Foundation currently plans to scale Ethereum. At the time of this writing the full spec is still not published. I wrote a detailed overview of Ethereum shard chains and comparison of their Beacon chain consensus to Near’s.
Near Protocol is also building sharding. Near team includes three ex-MemSQL engineers responsible for building sharding, cross-shard transactions and distributed JOINs, as well as five ex-Googlers, and has significant industry expertise in building distributed systems.
In this post I summarize the core ideas of blockchain sharding, on which both Near and majority of other sharded protocols are based. The subsequent post will outline more advanced topics in sharding.
The simplest Sharding, a.k.a. Beanstalk
Let’s start with the simplest approach to sharding, that we throughout this write-up will call a Beanstalk. This is also what Vitalik calls “scaling by a thousand altcoins” in this presentation.
In this approach instead of running one blockchain, we will run multiple, and call each such blockchain a “shard”. Each shard will have its own set of validators. Here and below we use a generic term “validator” to refer to participants that verify transactions and produce blocks, either by mining, such as in Proof of Work, or via a voting-based mechanism. For now let’s assume that the shards never communicate with each other.
The Beanstalk design, though simple, is sufficient to outline some major challenges in sharding.
Validator partitioning and Beacon chains
The first challenge is that with each shard having its own validators, each shard is now 10 times less secure than the entire chain. So if a non-sharded chain with X validators decides to hard-fork into a sharded chain, and splits X validators across 10 shards, each shard now only has X/10 validators, and corrupting one shard only requires corrupting 5.1% (51% / 10) of the total number of validators.
Which brings us to the second point: who chooses validators for each shard? Controlling 5.1% of validators is only damaging if all those 5.1% of validators are in the same shard. If validators can’t choose which shard they get to validate in, a participant controlling 5.1% of the validators is highly unlikely to get all their validators in the same shard, heavily reducing their ability to compromise the system.
Almost all sharding designs today rely on some source of randomness to assign validators to shards. Randomness on blockchain on itself is a very challenging topic and would deserve a separate blog post at some later date, but for now let’s assume there’s some source of randomness we can use.
Both the randomness and the validators assignment require computation that is not specific to any particular shard. For that computation, practically all existing designs have a separate blockchain that is tasked with performing operations necessary for the maintenance of the entire network. Besides generating random numbers and assigning validators to the shards, these operations often also include receiving updates from shards and taking snapshots of them, processing stakes and slashing in Proof-of-Stake systems, and rebalancing shards when that feature is supported. Such chain is called a Beacon chain in Ethereum and Near, a Relay chain in PolkaDot, and the Cosmos Hub in Cosmos.
Throughout this post we will refer to such chain as a Beacon chain. The existence of the Beacon chain brings us to the next interesting topic, the quadratic sharding.
Quadratic sharding
Sharding is often advertised as a solution that scales infinitely with the number of nodes participating in the network operation. While it is in theory possible to design such a sharding solution, any solution that has the concept of a Beacon chain doesn’t have infinite scalability. To understand why, note that the Beacon chain has to do some bookkeeping computation, such as assigning validators to shards, or snapshotting shard chain blocks, that is proportional to the number of shards in the system. Since the Beacon chain is itself a single blockchain, with computation bounded by the computational capabilities of nodes operating it, the number of shards is naturally limited.
However, the structure of a sharded network does bestow a multiplicative effect on any improvements to its nodes. Consider the case in which an arbitrary improvement is made to the efficiency of nodes in the network which will allow them faster transaction processing times.
If the nodes operating the network, including the nodes in the Beacon chain, become four times faster, then each shard will be able to process four times more transactions, and the Beacon chain will be able to maintain 4 times more shards. The throughput across the system will increase by the factor of 4 x 4 = 16 — thus the name quadratic sharding.
It is hard to provide an accurate measurement for how many shards are viable today, but it is unlikely that in any foreseeable future the throughput needs of blockchain users will outgrow the limitations of quadratic sharding. The sheer number of nodes necessary to operate such a volume of shards securely is orders of magnitude higher than the number of nodes operating all the blockchains combined today.
However, if we want to build future proof protocols, it might be worth starting researching solutions to this problem today. The most developed proposal as of now is exponential sharding, in which shards themselves are forming a tree, and each parent shard is orchestrating a series of child shards, while can itself be a child of some other shard.
Vlad Zamfir is known to be working on a sharding design that doesn’t involve a beacon chain; I worked with him on one of the prototypes, the detailed overview of which is here.
State Sharding
Up until now we haven’t defined very well what exactly is and is not separated when a network is divided into shards. Specifically, nodes in the blockchain perform three important tasks: not only do they 1) process transactions, they also 2) relay validated transactions and completed blocks to other nodes and 3) store the state and the history of the entire network ledger. Each of these three tasks imposes a growing requirement on the nodes operating the network:
The necessity to process transactions requires more compute power with the increased number of transactions being processed;
The necessity to relay transactions and blocks requires more network bandwidth with the increased number of transactions being relayed;
The necessity to store data requires more storage as the state grows. Importantly, unlike the processing power and network, the storage requirement grows even if the transaction rate (number of transactions processed per second) remains constant.
From the above list it might appear that the storage requirement would be the most pressing, since it is the only one that is being increased over time even if the number of transactions per second doesn’t change, but in practice the most pressing requirement today is the compute power. The entire state of Ethereum as of this writing is 100GB, easily manageable by most of the nodes. But the number of transactions Ethereum can process is around 20, orders of magnitude less than what is needed for many practical use cases.
Zilliqa is the most well-known project that shards processing but not storage. Sharding of processing is an easier problem because each node has the entire state, meaning that contracts can freely invoke other contracts and read any data from the blockchain. Some careful engineering is needed to make sure updates from multiple shards updating the same parts of the state do not conflict. In those regards Zilliqa is taking a very simplistic approach, which I analyze in this post.
While sharding of storage without sharding of processing was proposed, I’m not aware of any project working on it. Thus in practice sharding of storage, or State Sharding, almost always implies sharding of processing and sharding of network.
Practically, under State Sharding the nodes in each shard are building their own blockchain that contains transactions that affect only the local part of the global state that is assigned to that shard. Therefore, the validators in the shard only need to store their local part of the global state and only execute, and as such only relay, transactions that affect their part of the state. This partition linearly reduces the requirement on all compute power, storage, and network bandwidth, but introduces new problems, such as data availability and cross-shard transactions, both of which we will cover below.
Cross-shard transactions
Beanstalk as a model is not a very useful approach to sharding, because if individual shards cannot communicate with each other, they are no better than multiple independent blockchains. Even today, when sharding is not available, there’s a huge demand for interoperability between various blockchains.
Let’s for now only consider simple payment transactions, where each participant has account on exactly one shard. If one wishes to transfer money from one account to another within the same shard, the transaction can be processed entirely by the validators in that shard. If, however, Alice that resides on shard #1 wants to send money to Bob who resides on shard #2, neither validators on shard #1(they won’t be able to credit Bob’s account) nor the validators on shard #2 (they won’t be able to debit Alice’s account) can process the entire transaction.
There are two families of approaches to cross-shard transactions:
Synchronous: whenever a cross-shard transaction needs to be executed, the blocks in multiple shards that contain state transition related to the transaction get all produced at the same time, and the validators of multiple shards collaborate on executing such transactions. The most detailed proposal known to me is Merge Blocks, described here.
Asynchronous: a cross-shard transaction that affects multiple shards is executed in those shards asynchronously, the “Credit” shard executing its half once it has sufficient evidence that the “Debit” shard has executed its portion. This approach tends to be more prevalent due to its simplicity and ease of coordination. This system is today proposed in Cosmos, Ethereum Serenity, Near, Kadena, and others. A problem with this approach lies in that if blocks are produced independently, there’s a non-zero chance that one of the multiple blocks will be orphaned, thus making the transaction only partially applied. Consider the figure below that depicts two shards both of which encountered a fork, and a cross-shard transaction that was recorded in blocks A and X’ correspondingly. If the chains A-B and V’-X’-Y’-Z’ end up being canonical in the corresponding shards, the transaction is fully finalized. If A’-B’-C’-D’ and V-X become canonical, then the transaction is fully abandoned, which is acceptable. But if, for example, A-B and V-X become canonical, then one part of the transaction is finalized and one is abandoned, creating an atomicity failure. We will cover how this problem is addressed in proposed protocols in the second part, when covering changes to the fork-choice rules and consensus algorithms proposed for sharded protocols.
Note that communication between chains is useful outside of sharded blockchains too. Interoperability between chains is a complex problem that many projects are trying to solve. In sharded blockchains the problem is somewhat easier since the block structure and consensus are the same across shards, and there’s a beacon chain that can be used for coordination. In a sharded blockchain, however, all the shard chains are the same, while in the global blockchains ecosystem there are lots of different blockchains, with different target use cases, decentralization and privacy guarantees.
Building a system in which a set of chains have different properties but use sufficiently similar consensus and block structure and have a common beacon chain could enable an ecosystem of heterogeneous blockchains that have a working interoperability subsystem. Such system is unlikely to feature validator rotation, so some extra measures need to be taken to ensure security. Both Cosmos and PolkaDot are effectively such systems. This writeup by Zaki Manian from Cosmos provides detailed overview and comparison of the key aspects of the two projects.
Malicious behavior
You now have a good understanding of how sharding is implemented, including the concepts of the beacon chain, validator rotations and cross-shard transactions.
With all that information, there’s one last important thing to consider. Specifically, what adversarial behavior can malicious validators exercise.
Malicious Forks
A set of malicious validators might attempt to create a fork. Note that it doesn’t matter if the underlying consensus is BFT or not, corrupting sufficient number of validators will always make it possible to create a fork.
It is significantly more likely for more that 50% of a single shard to be corrupted, than for more than 50% of the entire network to be corrupted (we will dive deeper into these probabilities in the second part). As discussed above, cross-shard transactions involve certain state changes in multiple shards, and the corresponding blocks in such shards that apply such state changes must either be all finalized (i.e. appear in the selected chains on their corresponding shards), or all be orphaned (i.e. not appear in the selected chains on their corresponding shards). Since generally the probability of shards being corrupted is not negligible, we can’t assume that the forks won’t happen even if a byzantine consensus was reached among the shard validators, or many blocks were produced on top of the block with the state change.
This problem has multiple solutions, the most common one being occasional cross-linking of the latest shard chain block to the beacon chain. The fork choice rule in the shard chains is then changed to always prefer the chain that is cross-linked, and only apply shard-specific fork-choice rule for blocks that were published since the last cross-link. We will talk more about what fork-choice rules are, and provide an in-depth analysis of proposed fork-choice rules for sharded blockchains in the second part.
Approving invalid blocks
A set of validators might attempt to create a block that applies the state transition function incorrectly. For example, starting with a state in which Alice has 10 tokens and Bob has 0 tokens, the block might contain a transaction that sends 10 tokens from Alice to Bob, but ends up with a state in which Alice has 0 tokens and Bob has 1000 tokens.
In a classic non-sharded blockchain such an attack is not possible, since all the participant in the network validate all the blocks, and the block with such an invalid state transition will be rejected by both other block producers, and the participants of the network that do not create blocks. Even if the malicious validators continue creating blocks on top of such an invalid block faster than honest validators build the correct chain, thus having the chain with the invalid block being longer, it doesn’t matter, since every participant that is using the blockchain for any purpose validates all the blocks, and discards all the blocks built on top of the invalid block.
On the figure above there are five validators, three of whom are malicious. They created an invalid block A’, and then continued building new blocks on top of it. Two honest validators discarded A’ as invalid and were building on top of the last valid block known to them, creating a fork. Since there are fewer validators in the honest fork, their chain is shorter. However, in classic non-sharded blockchain every participant that uses blockchain for any purpose is responsible for validating all the blocks they receive and recomputing the state. Thus any person who has any interest in the blockchain would observe that A’ is invalid, and thus also immediately discard B’, C’ and D’, as such taking the chain A-B as the current longest valid chain.
In a sharded blockchain, however, no participant can validate all the transactions on all the shards, so they need to have some way to confirm that at no point in history of any shard of the blockchain no invalid block was included.
Note that unlike with forks, cross-linking to the Beacon chain is not a sufficient solution, since the Beacon chain doesn’t have the capacity to validate the blocks. It can only validate that a sufficient number of validators in that shard signed the block (and as such attested to its correctness).
I am aware of only two solutions to this problem, neither of which is really satisfactory today:
Have some reasonable mechanism that will alert the system if an attempt to apply the state transition incorrectly is made. Assuming that each shard is running some sort of BFT consensus, for as long as number of malicious validators in a particular shard is less than ⅔, at least one honest validator would need to attest to a block, and verify that the state transition function is applied correctly. If more than ⅔ of the nodes are malicious, they can finalize a block without a single honest node participating. Assuming that at least one node in the shard is not malicious, some mechanism is needed that would allow such nodes to monitor what blocks are being produced, and have sufficient time to challenge nodes with invalid state transition.
Have some information in the blocks that is sufficient to prove that the state transition is applied correctly but is significantly cheaper to validate than the actual application of the state transition function. The closest mechanism to achieve that is zk-SNARKs (though we don’t really need the “zk”, or zero-knowledge, part, a non-zk SNARK would be sufficient), but zk-SNARKs are notoriously slow to compute at this point.
Many protocols today assume that with proper validator rotation and a byzantine fault tolerant consensus neither forks nor invalid state transitions are possible. We will begin the subsequent Sharding 201 by addressing why this assumption is not reasonable.
Outro
With the above information you now know most of the important aspects of sharding, such as the concept of the Beacon chain, computation versus state sharding, and cross-shard transactions. Stay tuned for the second part with Sharding 201 which will dive deeper into attack prevention.
In the meantime be sure to join our Discord channel where we discuss all technical and non-technical aspects of Near Protocol, such as consensus, economics and governance:
https://discord.gg/nqAXT7h
We also recently open sourced our code, read more here, or explore the code on GitHub.
Make sure to follow Near Protocol on Twitter to not miss our future blog posts, including Sharding 201, and other announcements:
http://twitter.com/nearprotocol
http://twitter.com/AlexSkidanov
https://upscri.be/633436/
Huge thanks to Monica Quaintance from Kadena and Zaki Manian from Cosmos for providing lots of valuable early feedback on this post. |
---
id: royalty
title: Royalty
sidebar_label: Royalty
---
import {Github} from "@site/src/components/codetabs"
In this tutorial you'll continue building your non-fungible token (NFT) smart contract, and learn how to implement perpetual royalties into your NFTs. This will allow people to get a percentage of the purchase price when an NFT is sold.
## Introduction
By now, you should have a fully fledged NFT contract, except for the royalties support.
To get started, either switch to the `5.approval` branch from our [GitHub repository](https://github.com/near-examples/nft-tutorial-js/), or continue your work from the previous tutorials.
```bash
git checkout 5.approval
```
:::tip
If you wish to see the finished code for this _Royalty_ tutorial, you can find it on the `6.royalty` branch.
:::
## Thinking about the problem
In order to implement the functionality, you first need to understand how NFTs are sold. In the previous tutorial, you saw how someone with an NFT could list it on a marketplace using the `nft_approve` function by passing in a message that could be properly decoded. When a user purchases your NFT on the marketplace, what happens?
Using the knowledge you have now, a reasonable conclusion would be to say that the marketplace transfers the NFT to the buyer by performing a cross-contract call and invokes the NFT contract's `nft_transfer` method. Once that function finishes, the marketplace would pay the seller for the correct amount that the buyer paid.
Let's now think about how this can be expanded to allow for a cut of the pay going to other accounts that aren't just the seller.
### Expanding the current solution
Since perpetual royalties will be on a per-token basis, it's safe to assume that you should be changing the `Token` and `JsonToken` structs. You need some way of keeping track of what percentage each account with a royalty should have. If you introduce a map of an account to an integer, that should do the trick.
Now, you need some way to relay that information to the marketplace. This method should be able to transfer the NFT exactly like the old solution but with the added benefit of telling the marketplace exactly what accounts should be paid what amounts. If you implement a method that transfers the NFT and then calculates exactly what accounts get paid and to what amount based on a passed-in balance, that should work nicely.
This is what the [royalty standards](https://nomicon.io/Standards/NonFungibleToken/Payout) outlined. Let's now move on and modify our smart contract to introduce this behavior.
## Modifications to the contract
The first thing you'll want to do is add the royalty information to the structs. Open the `nft-contract/src/metadata.ts` file and add `royalty` to the `Token` and `JsonToken` structs:
```js
royalty: { [accountId: string]: number };
```
Second, you'll want to add `royalty` to the `JsonToken` struct as well:
<Github language="js" start="106" end="166" url="https://github.com/near-examples/nft-tutorial-js/blob/6.royalty/src/nft-contract/metadata.ts" />
### Internal helper function
**royaltyToPayout**
To simplify the payout calculation, let's add a helper `royaltyToPayout` function to `src/internal.ts`. This will convert a percentage to the actual amount that should be paid. In order to allow for percentages less than 1%, you can give 100% a value of `10,000`. This means that the minimum percentage you can give out is 0.01%, or `1`. For example, if you wanted the account `benji.testnet` to have a perpetual royalty of 20%, you would insert the pair `"benji.testnet": 2000` into the payout map.
<Github language="js" start="13" end="16" url="https://github.com/near-examples/nft-tutorial-js/blob/6.royalty/src/nft-contract/internal.ts" />
If you were to use the `royaltyToPayout` function and pass in `2000` as the `royaltyPercentage` and an `amountToPay` of 1 NEAR, it would return a value of 0.2 NEAR.
### Royalties
**nft_payout**
Let's now implement a method to check what accounts will be paid out for an NFT given an amount, or balance. Open the `nft-contract/src/royalty.ts` file, and modify the `internalNftPayout` function as shown.
<Github language="js" start="7" end="53" url="https://github.com/near-examples/nft-tutorial-js/blob/6.royalty/src/nft-contract/royalty.ts" />
This function will loop through the token's royalty map and take the balance and convert that to a payout using the `royaltyToPayout` function you created earlier. It will give the owner of the token whatever is left from the total royalties. As an example:
You have a token with the following royalty field:
```js
Token {
owner_id: "damian",
royalty: {
"benji": 1000,
"josh": 500,
"mike": 2000
}
}
```
If a user were to call `nft_payout` on the token and pass in a balance of 1 NEAR, it would loop through the token's royalty field and insert the following into the payout object:
```js
Payout {
payout: {
"benji": 0.1 NEAR,
"josh": 0.05 NEAR,
"mike": 0.2 NEAR
}
}
```
At the very end, it will insert `damian` into the payout object and give him `1 NEAR - 0.1 - 0.05 - 0.2 = 0.65 NEAR`.
**nft_transfer_payout**
Now that you know how payouts are calculated, it's time to create the function that will transfer the NFT and return the payout to the marketplace.
<Github language="js" start="55" end="121" url="https://github.com/near-examples/nft-tutorial-js/blob/6.royalty/src/nft-contract/royalty.ts" />
### Perpetual royalties
To add support for perpetual royalties, let's edit the `src/mint.ts` file. First, add an optional parameter for perpetual royalties. This is what will determine what percentage goes to which accounts when the NFT is purchased. You will also need to create and insert the royalty to be put in the `Token` object:
<Github language="js" start="7" end="64" url="https://github.com/near-examples/nft-tutorial-js/blob/6.royalty/src/nft-contract/mint.ts" />
### Adding royalty object to struct implementations
Since you've added a new field to your `Token` and `JsonToken` structs, you need to edit your implementations accordingly. Move to the `nft-contract/src/internal.ts` file and edit the part of your `internalTransfer` function that creates the new `Token` object:
<Github language="js" start="150" end="158" url="https://github.com/near-examples/nft-tutorial-js/blob/6.royalty/src/nft-contract/internal.ts" />
Once that's finished, move to the `nft-contract/src/nft_core.ts` file. You need to edit your implementation of `internalNftToken` so that the `JsonToken` sends back the new royalty information.
<Github language="js" start="10" end="37" url="https://github.com/near-examples/nft-tutorial-js/blob/6.royalty/src/nft-contract/nft_core.ts" />
Next, you can use the CLI to query the new `nft_payout` function and validate that it works correctly.
## Deploying the contract {#redeploying-contract}
As you saw in the previous tutorial, adding changes like these will cause problems when redeploying. Since these changes affect all the other tokens and the state won't be able to automatically be inherited by the new code, simply redeploying the contract will lead to errors. For this reason, you'll create a new sub-account again.
### Creating a sub-account
Run the following command to create a sub-account `royalty` of your main account with an initial balance of 25 NEAR which will be transferred from the original to your new account.
```bash
near create-account royalty.$NFT_CONTRACT_ID --masterAccount $NFT_CONTRACT_ID --initialBalance 25
```
Next, you'll want to export an environment variable for ease of development:
```bash
export ROYALTY_NFT_CONTRACT_ID=royalty.$NFT_CONTRACT_ID
```
Using the build script, build the deploy the contract as you did in the previous tutorials:
```bash
yarn build && near deploy --wasmFile build/nft.wasm --accountId $ROYALTY_NFT_CONTRACT_ID
```
### Initialization and minting {#initialization-and-minting}
Since this is a new contract, you'll need to initialize and mint a token. Use the following command to initialize the contract:
```bash
near call $ROYALTY_NFT_CONTRACT_ID init '{"owner_id": "'$ROYALTY_NFT_CONTRACT_ID'"}' --accountId $ROYALTY_NFT_CONTRACT_ID
```
Next, you'll need to mint a token. By running this command, you'll mint a token with a token ID `"royalty-token"` and the receiver will be your new account. In addition, you're passing in a map with two accounts that will get perpetual royalties whenever your token is sold.
```bash
near call $ROYALTY_NFT_CONTRACT_ID nft_mint '{"token_id": "approval-token", "metadata": {"title": "Approval Token", "description": "testing out the new approval extension of the standard", "media": "https://bafybeiftczwrtyr3k7a2k4vutd3amkwsmaqyhrdzlhvpt33dyjivufqusq.ipfs.dweb.link/goteam-gif.gif"}, "receiver_id": "'$ROYALTY_NFT_CONTRACT_ID'", "perpetual_royalties": {"benjiman.testnet": 2000, "mike.testnet": 1000, "josh.testnet": 500}}' --accountId $ROYALTY_NFT_CONTRACT_ID --amount 0.1
```
You can check to see if everything went through properly by calling one of the enumeration functions:
```bash
near view $ROYALTY_NFT_CONTRACT_ID nft_tokens_for_owner '{"account_id": "'$ROYALTY_NFT_CONTRACT_ID'", "limit": 10}'
```
This should return an output similar to the following:
```json
[
{
"token_id": "approval-token",
"owner_id": "royalty.goteam.examples.testnet",
"metadata": {
"title": "Approval Token",
"description": "testing out the new approval extension of the standard",
"media": "https://bafybeiftczwrtyr3k7a2k4vutd3amkwsmaqyhrdzlhvpt33dyjivufqusq.ipfs.dweb.link/goteam-gif.gif"
},
"approved_account_ids": {},
"royalty": {
"josh.testnet": 500,
"benjiman.testnet": 2000,
"mike.testnet": 1000
}
}
]
```
Notice how there's now a royalty field that contains the 3 accounts that will get a combined 35% of all sales of this NFT? Looks like it works! Go team :)
### NFT payout
Let's calculate the payout for the `"approval-token"` NFT, given a balance of 100 yoctoNEAR. It's important to note that the balance being passed into the `nft_payout` function is expected to be in yoctoNEAR.
```bash
near view $ROYALTY_NFT_CONTRACT_ID nft_payout '{"token_id": "approval-token", "balance": "100", "max_len_payout": 100}'
```
This command should return an output similar to the following:
```bash
{
payout: {
'josh.testnet': '5',
'royalty.goteam.examples.testnet': '65',
'mike.testnet': '10',
'benjiman.testnet': '20'
}
}
```
If the NFT was sold for 100 yoctoNEAR, josh would get 5, benji would get 20, mike would get 10, and the owner, in this case `royalty.goteam.examples.testnet` would get the rest: 65.
## Conclusion
At this point you have everything you need for a fully functioning NFT contract to interact with marketplaces.
The last remaining standard that you could implement is the events standard. This allows indexers to know what functions are being called and makes it easier and more reliable to keep track of information that can be used to populate the collectibles tab in the wallet for example.
:::info remember
If you want to see the finished code from this tutorial, you can checkout the `6.royalty` branch.
:::
:::note Versioning for this article
At the time of this writing, this example works with the following versions:
- near-cli: `3.0.0`
- NFT standard: [NEP171](https://nomicon.io/Standards/Tokens/NonFungibleToken/Core), version `1.0.0`
- Enumeration standard: [NEP181](https://nomicon.io/Standards/Tokens/NonFungibleToken/Enumeration), version `1.0.0`
- Royalties standard: [NEP199](https://nomicon.io/Standards/Tokens/NonFungibleToken/Payout), version `2.0.0`
:::
|
How Vietnam Became a Web3 Powerhouse
COMMUNITY
September 4, 2023
Asia is Web3’s engine room. According to a report by Emergen Research, the Web3 global market is expected to be worth $81.5 billion by 2030, growing at an annual rate of more than 40%. Asia is set to capture most of that growth.
That’s because the region has some of the highest adoption rates of Web3 technologies anywhere in the world. In Japan, more than five million people currently own cryptocurrency, the South Korean government became the first to directly invest in the metaverse, and almost 70% of people in Southeast Asia alone have used at least one metaverse-related tech in the last year.
One of the leaders in the region is Vietnam. In recent years, international investors have flooded into Vietnam and established funds specialising in blockchain – with capital inflows into non-fungible token (NFT) assets rising from 37 million USD to 4.8 billion USD in 2021.
It’s why the NEAR Foundation is one of the leading investors in Asia, thanks to its partnerships with the likes of Alibaba Cloud, and why it’s hosting its first major conference in Vietnam.
Taking place September 8-12 NEAR APAC will bring together more than 100 global blockchain leaders to discuss blockchain’s unlimited future through the latest blockchain technology updates, development trends, and how blockchain ecosystems drive Web3 mass adoption.
Vietnam on the rise
Vietnam has all the ingredients to become a Web3 super power. The country has
more than 400,000 skilled software engineers, produces 50,000 IT graduates annually, and has an IT labour force more than a million strong.
The nation’s software engineers rank at an impressive 8th place globally in terms of capability — 95% of which have bachelor’s degrees or higher in IT. It’s unsurprising then to hear that Vietnam now has 3,800 blockchain-related projects, 200 venture capital funds, and 100 project incubation funds to support the country’s startups.
Vietnam’s tech landscape is now full of startups and innovation hubs fostering creativity in Web3. Local incubators and accelerators provide mentorship, resources, and networking opportunities for Web3 entrepreneurs.
That growth also has government support. In 2021, Vietnamese Prime Minister Pham Minh Chinh greenlit the country’s “e-government” development strategy designed to create regulatory clarity for Web3 projects. Last year, the country launched its first official blockchain association, which is helping blockchain builders and business owners become a bigger part of the regulatory conversation.
Its chairman, Hoang Van Huay, has shared the Vietnam Blockchain Association’s commitment to “providing consultation on building legal framework, regulations, and standards in developing services and products on blockchain”. This commitment is part of a broader mission to accelerate the growth of blockchain technology in Vietnam.
It’s no wonder then that Vietnam ranks first in the Global Cryptocurrency Adoption Index released by Chainalysis, up from 10th place the year before.
Vietnam is on the rise, and the NEAR Foundation is here to support it. Join us at NEAR APAC on 8-12 September 2023 in Ho Chi Minh City. |
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
<Tabs groupId="nft-contract-tabs" className="file-tabs">
<TabItem value="NFT Primitive" label="Reference" default>
```bash
near call nft.primitives.near nft_mint '{"token_id": "1", "receiver_id": "bob.near", "token_metadata": {"title": "NFT Primitive Token", "description": "Awesome NFT Primitive Token", "media": "string"}}' --depositYocto 10000000000000000000000, --accountId bob.near
```
</TabItem>
<TabItem value="Paras" label="Paras">
```bash
near call x.paras.near nft_mint '{"token_series_id": "490641", "receiver_id": "bob.near"}' --depositYocto 10000000000000000000000 --accountId bob.near
```
:::note
In order to use `nft_mint` method of the `x.paras.near` contract you have to be a creator of a particular token series.
:::
</TabItem>
<TabItem value="Mintbase" label="Mintbase">
```bash
near call thomasettorreiv.mintbase1.near nft_batch_mint '{"num_to_mint": 1, "owner_id": "bob.near", "metadata": {}}' --accountId bob.near --depositYocto 10000000000000000000000
```
:::note
In order to use `nft_batch_mint` method of Mintbase store contract your account have to be a in the contract minters list.
:::
</TabItem>
</Tabs>
|
NEAR and SailGP Partner on Ground-Breaking New Sports DAO
COMMUNITY
March 25, 2022
NEAR is excited to announce a new partnership with SailGP, one of the world’s fastest-growing sailing events. As part of this ground-breaking multi-year partnership, SailGP will explore the sale of a new team to a DAO launched on the NEAR Protocol – a first-of-its-kind community engagement and activation platform in professional sports.
SailGP’s DAO will fuse professional sports with community-focused engagement, giving fans and crypto natives alike a new way to engage and gain access to their favorite teams, athletes, and related events.
Under the current SailGP Teams’ Participation Agreement, this new DAO team could join the SailGP starting line-up for Season 4 in 2023.
“We welcome NEAR to the SailGP family and we are looking forward to them participating in the most exciting racing on-water, featuring the world’s best sailors,” SailGP Founder Larry Ellison said.
How SailGP’s NEAR DAO will work
Like any other DAO, the SailGP team DAO will be a community project. Through proposals, the SailGP team DAO’s community members will help determine athlete selection, team management, commercialization options, operations, and overall team strategy.
The SailGP DAO will be a big departure from traditional sports team ownership structures. It will help reimagine sports communities, where fans have a say in how teams form and evolve.
Other NEAR and SailGP partnership initiatives
SailGP and NEAR will partner for a number of other new and exciting projects to help inspire a new generation of Web3 developers to build on NEAR.
Beyond DAOs, NEAR and SailGP will collaborate on integrated technology and research and development projects in events ticketing, NFT and gaming offerings, and mobile app integration. NEAR will also use SailGP’s global technology platform to develop real-world intellectual property and Web3 applications for fan engagement, data delivery and management, league management, and more.
NEAR will also create on-site experiences and activations at global SailGP events to help traditional industries transition to Web3 through NEAR’s simple, secure, and scalable blockchain platform. These events will be open to both new and existing NEAR community members.
“Our mission is to enable community-driven innovation to benefit people around the world, and this partnership is a fantastic example of exactly that,” said Marieke Flament, CEO of the NEAR Foundation, the non-for profit entity that oversees development on the NEAR blockchain.
“We are ushering in a brand-new era of entertainment by combining sports and technology to elevate the fan experience to a level that has never been seen before, and we’re proud to be leading the way alongside a like-minded organization that shares our core values and vision.”
IMG, SailGP’s global agency for sponsorship, media rights and host venue rights, helped facilitate NEAR’s partnership with SailGP. (IMG is part of Endeavor, a strategic investor in SailGP.)
“This deal presents an exciting opportunity to change how fans can directly influence and participate in the sports they love,” said Mark Shapiro, President of Endeavor. “We are proud to have brokered this ground-breaking partnership which is the culmination of what happens when sport, technology and entertainment collide.”
|
---
sidebar_label: "Intro to Indexers"
---
# Introduction to Indexers
Here you will find everything you need to know in order to familiarize yourself with the concept of indexers and even build your own one.
:::info Disclaimer
The explanation on this page assumes you have a certain level of understanding of the blockchain technology.
:::
### Blockchains and their nature
Blockchain data is optimized for serialized **writes**, one block at a time, as the chain is being created. Querying the blockchain for data about a specific block or account is fairly straightforward or a "narrow" query. However, querying data across many blocks can be cumbersome because we have to aggregate results from multiple single-block queries. Therefore, we can consider these *"wide" queries*.
Given the fact that a blockchain itself is a distributed database, and a smart contract (decentralized application, dApp) is an application that runs on a virtual machine inside a blockchain, we need to understand that smart contracts should *not* be considered as a "backend". While some applications might consist only of smart contracts, building a dApp with only smart contracts, in most cases, is not possible.
Smart contracts are limited in terms of interactions. By "interactions" we mean things that are very common in the real world, like user notifications, integration with third-party applications, etc.
However, the nature of a blockchain is that it *must* be deterministic. A critical feature of a blockchain is that it knows the state at a given time, and for blockchains that time unit is a block. Think of them as being snapshots. A blockchain does snapshots of its state on every block. We as users can call smart contracts for a specific block, and the blockchain provides guarantees that execution will always produce the same result for the same block any time we call it.
The deterministic nature of a blockchain closes it from external (off-chain) variables. It is totally impossible to perform a call to an API from within a smart contract. A blockchain and a smart contract are closed off from the external (off-chain) world.

Blockchains are great at providing a way to apply the requested changes to the state in a decentralized manner. However, in order to observe the changes, you need to actively pull the information from the network.
Instead of abstract explanations let's look at an example.
:::note Example dApp
Say, we have a smart contract that sells e-books. Once a user buys a book we want to send them a copy via email.
:::
The dApp has a helper deployed somewhere off-chain, and this helper has code that can send an email with a copy of an e-book. But how would we trigger the helper?
### Getting the data from a blockchain from the external world
NEAR blockchain implements a [JSON-RPC endpoint](https://docs.near.org/api/rpc/introduction) for everyone to interact with the blockchain. Through the JSON-RPC API users can call smart contracts triggering them to be executed with given parameters. Also, users can view the data from the blockchain.
So, continuing with our example we can make our helper pull a [Block](https://docs.near.org/api/rpc/block-chunk#block) every second, then pull all the [Chunks](https://docs.near.org/api/rpc/block-chunk#chunk) and analyze the Transactions included in the Block to check if there is a transaction to our smart contract with "buy an e-book" function call. If we observe such a Transaction, we need to ensure it is successful, so we don't send the e-book to a user whose "buy e-book" Transaction failed.
After the process is complete we can trigger the helper's code to send the user an email with the e-book they bought.
This approach is so-called *pull model* of getting the data. There is nothing wrong with this approach, but sometimes you might find it is not the most comfortable or reliable approach.
Also, not all the data is available through the JSON-RPC. *Local Receipts* for example are not available through the JSON-RPC, because they are not stored in NEAR node's internal database.
### Indexer
A blockchain indexer is an implementation of the *push model* of getting the data. Instead of actively pulling the data from the source, your helper waits for the data to be sent to it. The data is complete and so the helper can start analyzing it immediately; ideally the data is complete enough to avoid additional pulls to get more details.
Getting back to our example, the helper becomes **an indexer** that receives every *Block*, along with **Chunks**, **Transactions** with its statuses, etc. In the same way the helper analyzes the data and triggers the code to send the user an email with the e-book they bought.

:::info An indexer concept
An indexer listens to the *stream of data as it's being written on chain* and can then be immediately filtered and processed to detect interesting events or patterns.
:::
## Indexers and "wide" queries
The term *"wide" queries* was mentioned in the beginning of this document. Here's a recap:
:::note "Wide" queries definition
To query data across many blocks requires the aggregation of results from multiple single-block queries. We can consider these aggregates as coming from *"wide" queries*.
:::
Because indexers listen to the *stream of data* from the blockchain and the data can be immediately filtered and processed according to defined requirements, they can be used to simplify the "wide" queries execution. For example, a stream of data can be written to a permanent database for later data analysis using a convenient query language like SQL. That is what [Indexer for Explorer](/tools/indexer-for-explorer) is doing.
Another example that highlights the need for a "wide query" is when you use a seed phrase to recover one or more accounts. Since a seed phrase essentially represents a signing key pair, the recovery is for all accounts that share the associated public key. Therefore, when a seed phrase is used to recover an account via [NEAR Wallet](https://wallet.near.org), the query requires that all accounts with a matching public key are found and recovered. [NEAR Indexer for Explorer](/tools/indexer-for-explorer) is storing this data in a permanent database and this allows [NEAR Wallet](https://wallet.near.org) to perform such "wide queries". This is impossible to achieve using JSON-RPC only.
## Summary
We hope this article gives you an understanding of the Indexer concept. Also, we hope now you can easily decide whether you need an indexer for your application.
## What's next?
We encourage you to learn more about the [Lake Indexer project](/tools/realtime#near-lake-indexer). Please, proceed to [Tutorials](/build/data-infrastructure/lake-framework/near-lake-state-changes-indexer) section to learn how to build an indexer on practice.
Alternatively, there are a few other third-party indexers that are tightly integrated with the NEAR ecosystem. You can review all of your data sourcing options (including The Graph, Pagoda, Pipespeak, and SubQuery) under [data tools](/concepts/data-flow/data-storage#data-tools).
|
NEAR & Gaming: The Future of Gaming on Web3
COMMUNITY
August 22, 2022
Most people when asked why they play games will give a simple answer: because games are fun. And though “fun” might mean different things to different people, with Web3 gaming, at least in its present form, “fun” is only sometimes part of the equation.
“We tend to conflate games with fun, but the thing is, a game doesn’t necessarily have to be fun to be engaging,” says Chase Freo, CEO and Co-founder of OP Games. “There are a lot of different games out there that evoke different types of emotions.”
Though many gamers have a keen sense of what they enjoy and want to see more of, Web3 gaming’s direction will be determined not only by what is fun, but also by what developers and gamers realize is possible through experimentation.
Freo compares Web3 gaming to couture fashion season, when designers and runway models often show styles that stretch the limits of taste and the imagination. Think enormous hats, clothes made of garbage, and costumes that would seem extreme even at Burning Man. This couture fashion isn’t meant for the street—it’s made to see what’s possible.
In Web3 gaming, the goal isn’t necessarily to make or viral iconic games, but to plant the seeds for what games might one day be able to do. This starts with the technology itself.
Web2.5: The bridge to Web3
“A lot of times people sacrifice player experience to have a Web3 spin to it—that makes sense,” says Freo, who says gaming needs developers willing to experiment and try new things to evolve the ecosystem. “For example, bring[ing] game states on-chain [is] not optimal…but you kind of want to push it that way, so that you’ll see exactly where you can draw the line.”
Freo explains that there are generally two camps of developers: Web2 game designers who focus on gameplay, and devs who really push the envelope with blockchain but sacrifice playability in the process.
“Most games in Web3 are actually a combination of Web2 and Web3, so it’s like Web2.5,” says Freo. “[They combine] aspects of what works on the blockchain [and] everything else is centralized because it works better.”
Hugo Furneaux and Jon Hook at playEmber, a game-fi middleware company that brings Web3 to Web2 mobile games, echo Freo’s “Web2.5” view. Furneaux, playEmber’s CEO, describes the platform as “an amalgamation” of Web2 and Web3, where developers make extremely fun games while on-ramping Web2 users into Web3. They simply play games they already know and love with Web3 features that are low-key.
“There’s an added element now, which makes it more fun, where you can just put in your email [and] we do all the rest in the background,” he says. “And it sets up your NEAR wallet and you’re playing, effectively, a Web3 game but as a Web2 user.”
Getting gamers to board the Web3 train
At the forefront of Web3 gaming is NEAR’s Human Guild. Created in early 2021 by Sasha Hudzilin and Vlad Grichina, both early builders on NEAR, their approach is simple: easy onboarding and gamers earning by doing what they love.
“The mission is to help people earn in crypto and join the online economy,” says Hudzilin.
Human Guild also helps game designers develop their ideas, launch on mainnet, improve their products, and generally expand an already strong, supportive community of game developers. This makes Human Guild a vital resource within the NEAR ecosystem.
“[We] provide support starting with the concept of the game, designing economic upside for the community to get involved, DAO creation, bootstrap community engagement, consider foundries, share initial MVP with the community, and involve influencers,” says Hudzilin.
“We know Web3 can be difficult to navigate,” he adds. “So, we want to make sure people are not discouraged and can get the best out of this space.”
Indie versus AAA game studios in Web3
Currently the most popular Web3 games feature around 200,000 active wallets, says Hudzilin, and there are only a few of them. By comparison, Web2 mobile games can have over 100 million users, and in some cases one billion users. Truly, these early days demand that designers think differently about what games are and what they can be.
Perhaps because of Web3 games’ relatively low numbers, most of the creativity in game development comes from smaller indie studios. Not unlike the music, film, or book publishing industries, bigger budget games from AAA studios tend to be more formulaic (and less likely to take risks). This makes some sense, as AAA studios are often more pressed to turn a profit and follow previously successful models. However, Web3’s low market saturation does have its advantages.
“If you do launch, you’re almost bound to succeed,” says Hudzilin, who notes the bar for success is about 10k users giving the game a shot. “Especially in an ecosystem like NEAR where very few things have gone live so far.”
Human Guild is starting to focus more on smaller indie game developers, as they can deliver on smaller budgets with more creative spark. The idea is that this creativity will carry over into the future of Web3 gaming. Crypto games are labors of love and passion projects. Some developers even sacrifice career stability for their projects.
“Sometimes they live below the poverty line, which is kind of crazy because they’re very talented developers,” says Hudzilin. “And so we work with some of them.”
While most pure Web3 games don’t have mass market appeal, many companies are trying hard to make that happen. PlayEmber, for instance, is all about mass market appeal with their “hyper casual” gaming experiences that look very much like Web2 mobile games. With Ember Coin, playEmber offers a “play and earn” model where gamers don’t even need to think about earning cryptocurrency. Their goal is ambitious: to onboard a million new crypto wallets within a year once their games are live on EmberCoin.
Bridges to the physical world
There is also a real hunger to see games interfacing with the physical world. Endlesss, a live collaborative music creation platform inspired by game mechanics, recently showcased one possibility at NFT.NYC: a MIDI-enabled arcade game. Although arcades are niche, this experience opened a door to other Web3 physical integrations and in-person social interactions.
For playEmber’s Jon Hook, the Web3 bridge to the physical world could feature partnerships with big brands for retail experiences.
“Imagine partnering with Nike: you’re playing a game and hidden within the game are magic loot boxes,” he muses. “When you find one, it actually unlocks sneakers. It’s a little NFT so that when you go into the Nike store, you redeem it. It’s kind of like gamifying retail.”
This is, of course, in addition to designing and buying NFT avatars and using them across a gaming or Web3 ecosystem. Just like in the physical world, Furneaux envisions an experience where “you change moods, you change styles, you change clothes, so why shouldn’t you be able to change your NFT to reflect that too?”
NFTs are also useful for re-engagement. Jon Werthen Jr. at ARterraLabs envisions players earning in-game NFTs, then using them as an entry fee for gaming tournaments or a token to redeem real-world prizes.
A tapestry of visionaries
One thing that Web3 gaming has going for it is the abundance of visionaries. No doubt a crucial ingredient for crypto gaming’s future.
Human Guild’s Vlad Grichina, for instance, imagines a world where massive multiplayer games keep running forever on the blockchain—especially games from the 2000s and 2010s that disappeared because server costs were just too high for game studios. How? A separate group of validators would essentially run that part of the blockchain.
“As long as a community is interested in this kind of game, it should continue,” Hudzilin says.
Futuristic possibilities aside, currently Human Guild is gearing up for NEARCON where the NEARCON Gaming Lounge will have up to 20 playable versions of games. This is as much about research as it is entertainment, as the Human Guild will use NEARCON metrics to measure things like retention.
Despite the visionary nature of many Web3 game developers, there is little certainty about what it will look like in the future. Will personal computers still dominate Web3 games? Can mobile developers break past gatekeepers like Apple’s App Store? Will consoles jump aboard? What about virtual reality? It is likely to be some combination of all of these, but only time will tell.
It’s likely that Web3 game developers, on NEAR’s carbon neutral protocol or others like it, will redefine what is possible. This is something that current and future gamers alike can look forward to. |
Near at ETHDenver Hackathon Bounty Winners
NEAR FOUNDATION
March 13, 2023
For the 2023 edition of ETHDenver, the Near ecosystem offered a number of bounties across a range of Web3 verticals, including DeFi, Content, Search, Social Media, Interoperability, Smart Contract History, and more. There were 3 main prize pools from Aurora, Proximity, and Developer Governance (DevGov).
Congratulations to all Near winners. who received over 30k in USDC! Let’s have a look at the winning projects of the Near at ETHDenver bounties.
Aurora Bounty Winners
Open Aurora Bounty
Winner – StakingPool Aurora
Implements a liquid staking protocol around Aurora Plus staking. Users will deposit AURORA and receive a share in $stAUR tokens, which distributes the APY rewards. $stAUR could be immediately converted with a fee or wait for a delayed unstake.
Runner Up – Geni
AI-powered specifications generator of security tests for smart contracts.
Runner Up – Demeter
A lending platform that offers yield advancements, i.e., self-paying loans. Borrowers’ collateral is invested in RWA protocols, and the yield pays off debt.
Proximity Bounty Winners
Deploy a Decentralized Frontend Component
Winner – BosWrap
BosWrap used the Near Blockchain Operating System to build a decentralized widget for wrapping/unwrapping ETH.
Runner Up – ABI BOS Widget
A solidity explorer widget that allow users to explore any smart contract for a given ABI. You can get ABI from Etherscan website.
Runner Up – Bossed Up
A project focusing on Ethereum frontends, Blockchain Operating System improvements, and NEAR Social widgets that interact with NEAR contracts.
Runner Up – Atmosph3re
A dApp for reducing the homeless crisis in the United States by giving those living on the streets a decentralized identity, mentoring and community support, and funds for spending on their basic needs to help them thrive.
Runner Up – EthDenver2023UWCA
A dApp on NeaR’s new frontend with BOS.GG. Integrates with Rocketpool to offer quick swaps on Ethereum.
DevGov Bounty Winners
Extensive Developer Profile Widget
Winner – Developer Profile
A recreation of Github profile for NEAR social developers in the Blockchain Operating System.
Fuzzy Search Widget / Promoted Posts Banner Widget / Top Posts Widget / Post History with Diff Widget
Winner – Near.Social DevGovGigs Improvements
A new Developer Governance Gigs interaction experience for Near.Social. Features: 1) Fuzzy Searching that can handle typos, 2) Promote posts and Promoted Post viewer, 3) Hottest Posts viewer, and 4), Post editing history viewer.
Near.Social Bots Coded in Javascript
Winner – JS Bot Widget
Verifiable RPC Replies
Winner – Near Verifiable RPC Endpoints for Viewing Access Keys
Adds proof generation support to RPC read access key endpoints on the NEAR protocol to help pave the road for light client support.
|
Taco Labs and NEAR Foundation to Give Shopify Retailers Next-Gen Customer Loyalty Programs
NEAR FOUNDATION
May 5, 2023
E-commerce is entering a new era of customer loyalty as Taco Labs and NEAR Foundation join forces to revolutionize the way 5.6 million Shopify retailers engage and retain customers. This innovative partnership fosters personalized loyalty experiences that deepen connections between brands and consumers, transcending traditional loyalty program limitations.
By merging Taco Labs’ inventive platform with NEAR’s speedy, carbon-neutral blockchain, Shopify retailers can develop captivating loyalty programs while overcoming Web3 adoption challenges, such as slow transactions and high fees. The alliance paves the way for engaging multiplayer experiences, token-gated access, and exclusive benefits that transform customer retention and brand affinity.In this new loyalty landscape, both brands and consumers stand to benefit from this groundbreaking collaboration.
Taco Labs and NEAR: Loyalty reinvention unwrapped
As e-commerce continues to evolve, customer loyalty programs must adapt to remain relevant and effective. Traditional loyalty programs often struggle to build meaningful connections with customers, resulting in missed opportunities for improved retention and brand loyalty. Taco Labs and NEAR Foundation have partnered to address this issue, offering groundbreaking solutions to Shopify retailers.
The Starbucks case study illustrates how these solutions can foster unique and engaging loyalty experiences. With its recently unveiled Odyssey initiative, Starbucks created a Web3 loyalty program centered on gamification and real-life participation. Customers engage in challenges — like trying new menu items — to earn digital collectible stamps (NFTs). These stamps can be traded, and provide access to exclusive perks like limited-edition token-gated products, virtual classes, and trips to Starbucks roasteries or coffee farms.
Similar to Starbucks, Taco Labs is harnessing Web3 to create innovative and captivating customer experiences and connections. The Taco App is doing this through quest gamification, membership tiers, reward points, and referral programs. These experiences are delivered swiftly, seamlessly, and at scale using the NEAR Protocol.
Taco Labs and NEAR Foundation will further facilitate innovative loyalty approaches, incentivizing brand-valuable behavior, enhancing customer engagement, and transforming the e-commerce loyalty landscape.
Streamlining loyalty with no-code solutions
Taco Labs’ comprehensive platform addresses both immediate business needs and fosters long-term growth. It enables brands to scale their loyalty programs without incurring high costs and adapts to various business models, making the platform suitable for diverse industries and market segments.
A key strength of Taco Labs is its seamless integration with existing systems, connecting easily with popular e-commerce platforms like Shopify and in-store Point of Sale (POS) systems. This cross-channel integration ensures a cohesive and immersive customer experience across online and offline touchpoints.
Taco’s no-code approach streamlines the process for brands, marketers, and agencies. The end-to-end workflow doesn’t require specialized technical knowledge or developers, allowing businesses to focus on building meaningful connections with customers.
In the Web3 world, privacy and data security are crucial. Taco addresses this by giving brands control over customer data, which is stored on a decentralized, transparent network. This ensures security and privacy while enabling brands to leverage valuable information for decision-making.
Combining Web3 and the user-friendly NEAR Protocol, Taco Labs revolutionizes customer loyalty programs. Taco’s scalable, adaptable, and secure features enable businesses to create engaging experiences that retain customers and foster lasting connections, differentiating loyalty programs in a competitive market.
Spicing up Shopify loyalty
Taco Labs and NEAR Foundation will provide innovative solutions for Shopify retailers to enhance customer loyalty programs. By using Web3 technology, businesses can launch exclusive, early-access products quickly. Integration with Shopify delivers Web3 benefits while preserving Web2’s convenience and user experience.
With Nike’s successful CryptoKicks NFT campaign as another example, Taco Labs enables Shopify retailers to create similar experiences. Businesses can launch tiered NFTs to crowdfund new product lines, offering lifelong perks to early supporters, like monthly loyalty tokens for exclusive products and offers. This encourages early adoption and strengthens customer relationships.
Taco Labs’ platform promotes brand-valuable behavior by allowing customers to buy, sell, and gift the NFTs they’ve received. This generates a dynamic ecosystem of gifting and trading, attracting new customers without costly marketing. The platform’s blockchain foundation enables comprehensive data analytics, providing customer insights while respecting privacy.
A key advantage of Taco Labs and NEAR Foundation’s collaboration is user control over data. Customers can choose to share information or maintain privacy, fostering trust and a positive experience. With data stored on a public blockchain, no third party can revoke access, ensuring businesses retain full control over customer relationships.
Taco Labs and NEAR offer Shopify retailers a powerful, flexible solution to boost customer loyalty and engagement by combining Web3 and Web 2 features. This partnership presents an excellent growth opportunity for businesses in the rapidly evolving digital landscape.
The collaboration ushers in a new era of customer loyalty, empowering Shopify retailers to harness Web3 capabilities. By seamlessly integrating blockchain technology with existing platforms, businesses can create tailored, engaging experiences fostering lasting customer connections.
From token-gated products to comprehensive analytics, this partnership enables unique, sustainable loyalty programs, blending the strengths of Web3 and Web2 technologies. As the future of customer engagement unfolds, Taco Labs and the NEAR ecosystem are at the forefront. |
---
title: Getting Started
description: Your quick-start guide to NEAR Protocol and the NEARverse
sidebar_position: 0
---
# Getting Started
:::info on this page
* What is NEAR Protocol?
* Understanding NEAR's token utility
* Why you should build on NEAR
:::
This [open-source](https://github.com/near/wiki), collaborative wiki connects you to information about everything the protocol has to offer including usage, infrastructure, opportunities, and guilds.
Contributors to this Wiki include the NEAR Community and members of the NEAR Foundation team. NEAR Wiki endeavors to keep up to date information, both evergreen and otherwise.
## What is NEAR Protocol?
NEAR is a simple, scalable, and secure blockchain platform designed to provide the best possible experience for developers and users alike, which is necessary to bridge the gap to mainstream adoption of decentralized applications and the broader Web3 ecosystem. NEAR is completely carbon neutral as certified by [South Pole](https://near.org/blog/near-climate-neutral-product/).
Unlike other blockchains, the NEAR Protocol network has been built from the ground up to be the easiest in the world to adopt for both developers and their end-users while still providing the scalability necessary to serve those users.
Specifically, NEAR is designed to make it easier to:
**Build decentralized applications**-- whether you're a seasoned blockchain developer or only used to building with "traditional" web or app concepts, NEAR makes it easy to build the next generation of decentralized applications.
**Onboard users with a smooth experience**-- even if they have never used crypto, tokens, keys, wallets, or other blockchain artifacts.
**Scale your application seamlessly**-- the underlying platform automatically expands capacity via sharding without additional costs or effort on your part.
## What is the NEAR Token and How Can I Use It?
NEAR is the native token of the NEAR blockchain. It has a variety of use cases:
**Transaction fees** - All transactions which occur on the NEAR blockchain must be paid for using NEAR. Due to the proof-of-stake (PoS) and scalable nature of the chain, these transaction fees are often very low.
**Staking** - Fundamental to PoS ecosystems, NEAR can be delegated to validators (staked) to earn NEAR rewards.
**Acquiring a validator seat** - To become a validator on the NEAR blockchain a minimum amount of NEAR is required.
**dApps** - Those building on the NEAR ecosystem can, and have, choose to leverage the NEAR token in a number of ways expanding on its utility.
## Why NEAR?
You may have heard of distributed computing, cooperative cloud databases, or peer-to-peer networks--all of which play a role in blockchains.
Currently, most web-services utilize a single server and a single database to process your requests and provide information. This infrastructure is usually managed by an individual entity who treats all of their data processing like a black box: the request goes in, something happens, and the user receives an output.
While the company may rely on third parties to verify those claims, the user will never be able to verify what happened in the black box. This system relies on trust between users and companies.
NEAR is similar in principle to the “cloud-based” infrastructure that developers currently use to build applications, except that the cloud is no longer controlled by a single company running a giant data center — that data center is actually made up of all the people around the world who are operating nodes on the decentralized network. Instead of a “company-operated cloud,” it's a “community-operated cloud.”
NEAR Protocol is considered a “base-layer blockchain,” or a layer-one, meaning that it’s on the same level of the ecosystem as projects like Ethereum or Cosmos. That means everything in the ecosystem is built on top of the NEAR blockchain, including your decentralized application (dApp).
:::note link
Interested in learning more? Check out the [NEAR Website](https://near.org).
:::
_To contribute to this wiki, see the_ [_Contributing page_](contribute/wiki-contributing.md)
This wiki is a great resource to start learning about NEAR.
The next step in your NEAR journey, if you haven't yet, is to [create your own NEAR Wallet](overview/tokenomics/creating-a-near-wallet.md).
Your NEAR Wallet is your key to the world of dApps and Web3 on NEAR. |
Send NEAR to Anyone with NEAR Drops
CASE STUDIES
July 1, 2020
As important as scalability is (just take a look at ETH Gas Station to see how important), there is more to a quality user experience than transactions-per-second.
We want NEAR to be easy to use for both developers and end users: user accounts have human readable names, developers can pay for fees on behalf of their users, and smart contracts can be built with popular programming languages.
And now, users and apps can send NEAR to anyone, whether they have a NEAR account or not.
A Better Way to Onboard Users
Introducing NEAR Drops: a feature that makes onboarding users to NEAR much simpler.
In essence, a NEAR drop lets you send a link to whoever you want with a certain amount of $NEAR attached to it. This allows:
App developers to onboard users and pay for their NEAR account
Users to send $NEAR to their friends, whether they have an account or not
For app developers, NEAR Drops substantially improve the user onboarding process. Simply send new users a NEAR Drop referral link, they’ll be redirected to the NEAR Wallet’s account creation page, and they can create an account called [user].near. That’s it. They now have a NEAR account.
On NEAR, a user account is similar to a smart contract account on Ethereum, plus an Ethereum Name Service (ENS) name. This means that users get a readable account name and advanced key management, but it also means that creating a user account requires some $NEAR. With NEAR Drops, users can create an account without needing to have any $NEAR themselves.
How NEAR Drops Work
There are two main functions in the NEAR Drop contract: send and create_account_and_claim. The send function is called to initiate the NEAR drop, and requires a $NEAR deposit, which will pay for the account creation costs and be transferred to the end recipient. The function takes the public key of a public/private keypair as an argument, adds the key to the NEAR Drop contract as an authorized access key, and records the $NEAR deposit as owned by the public key.
Next, the developer sends the private key to the end user, generally in the form of a referral link. The user will go through the NEAR Wallet onboarding process and choose an account name. In the Wallet, a new public/private keypair is generated, and the Wallet calls the create_account_and_claim function with the user’s selected account name. This transaction is signed by the private key received in the link from the previous step, as only this key is authorized to call the NEAR Drop contract and retrieve the deposited $NEAR.
In turn, create_account_and_claim will recover the public key from the signature, create an account with the chosen account name, add the new public key (of which the private key is only known by the recipient) as the full access key for the new account, fund it with the $NEAR (less transaction fees), and remove the original public key as an access key on the contract.
And Voila! The user now has a non-custodial, funded NEAR account.
Try it Out
You can try NEAR Drops out for yourself here: https://near-drop-mainnet.onrender.com/
After logging in:
Select “Create New NEAR Drop”.
Enter the amount of NEAR you’d like to add to the link (try 50)
Authorize the transaction
Copy the link, and send it to a friend! (Or open it yourself)
If you want to dive even deeper into the technical details, the code for NEAR drops is publicly available in the near-linkdrop repository (from line 48).We believe that NEAR drops significantly reduces the barriers of entry to the NEAR platform and are excited to see our community start using it! |
# EpochManager
## Finalizing an epoch
At the last block of epoch `T`, `EpochManager` computes `EpochInfo` for epoch `T+2`, which
is defined by `EpochInfo` for `T+1` and the information aggregated from blocks of epoch `T`.
`EpochInfo` is all the information that `EpochManager` stores for the epoch, which is:
- `epoch_height`: epoch height (`T+2`)
- `validators`: the list of validators selected for epoch `T+2`
- `validator_to_index`: Mapping from account id to index in `validators`
- `block_producers_settlement`: defines the mapping from height to block producer
- `chunk_producers_settlement`: defines the mapping from height and shard id to chunk producer
- `hidden_validators_settlement`: TODO
- `fishermen`, `fishermen_to_index`: TODO. disabled on mainnet through a large `fishermen_threshold` in config
- `stake_change`: TODO
- `validator_reward`: validator reward for epoch `T`
- `validator_kickout`: see [Kickout set](#kickout-set)
- `minted_amount`: minted tokens in epoch `T`
- `seat_price`: seat price of the epoch
- `protocol_version`: TODO
Aggregating blocks of the epoch computes the following sets:
- `block_stats`/`chunk_stats`: uptime statistics in the form of `produced` and `expected` blocks/chunks for each validator of `T`
- `proposals`: stake proposals made in epoch `T`. If an account made multiple proposals, the last one is used.
- `slashes`: see [Slash set](#slash-set)
### Slash set
NOTE: slashing is currently disabled. The following is the current design, which can change.
Slash sets are maintained on block basis. If a validator gets slashed in epoch `T`, subsequent blocks of epochs `T` and
`T+1` keep it in their slash sets. At the end of epoch `T`, the slashed validator is also added to `kickout[T+2]`.
Proposals from blocks in slash sets are ignored.
It's kept in the slash set (and kickout sets) for two or three epochs depending on whether it was going to be a validator in `T+1`:
- Common case: `v` is in `validators[T]` and in `validators[T+1]`
- proposals from `v` in `T`, `T+1` and `T+2` are ignored
- `v` is added to `kickout[T+2]`, `kickout[T+3]` and `kickout[T+4]` as slashed
- `v` can stake again starting with the first block of `T+3`.
- If `v` is in `validators[T]` but not in `validators[T+1]` (e.g. if it unstaked in `T-1`)
- proposals from `v` in `T` and `T+1` are ignored
- `v` is added to `kickout[T+2]` and `kickout[T+3]` as slashed
- `v` can make a proposal in `T+2` to become a validator in `T+4`
- If `v` is in `validators[T-1]` but not in `validators[T]` (e.g. did slashable behavior right before rotating out)
- proposals from `v` in `T` and `T+1` are ignored
- `v` is added to `kickout[T+2]` and `kickout[T+3]` as slashed
- `v` can make a proposal in `T+2` to become a validator in `T+4`
## Computing EpochInfo
### Kickout set
`kickout[T+2]` contains validators of epoch `T+1` that stop being validators in `T+2`, and also accounts that are not
necessarily validators of `T+1`, but are kept in slashing sets due to the rule described [above](#slash-set).
`kickout[T+2]` is computed the following way:
1. `Slashed`: accounts in the slash set of the last block in `T`
2. `Unstaked`: accounts that remove their stake in epoch `T`, if their stake is non-zero for epoch `T+1`
3. `NotEnoughBlocks/NotEnoughChunks`: For each validator compute the ratio of blocks produced to expected blocks produced (same with chunks produced/expected).
If the percentage is below `block_producer_kickout_threshold` (`chunk_producer_kickout_threshold`), the validator is kicked out.
- Exception: If all validators of `T` are either in `kickout[T+1]` or to be kicked out, we don't kick out the
validator with the maximum number of blocks produced. If there are multiple, we choose the one with
lowest validator id in the epoch.
4. `NotEnoughStake`: computed after validator selection. Accounts who have stake in epoch `T+1`, but don't meet stake threshold for epoch `T+2`.
5. `DidNotGetASeat`: computed after validator selection. Accounts who have stake in epoch `T+1`, meet stake threshold for epoch `T+2`, but didn't get any seats.
### Processing proposals
The set of proposals is processed by the validator selection algorithm, but before that, the set of proposals is adjusted
the following way:
1. If an account is in the slash set as of the end of `T`, or gets kicked out for `NotEnoughBlocks/NotEnoughChunks` in epoch `T`,
its proposal is ignored.
3. If a validator is in `validators[T+1]`, and didn't make a proposal, add an implicit proposal with its stake in `T+1`.
2. If a validator is in both `validators[T]` and `validators[T+1]`, and made a proposal in `T` (including implicit),
then its reward for epoch `T` is automatically added to the proposal.
The adjusted set of proposals is used to compute the seat price, and determine `validators`,`block_producers_settlement`,
`chunk_producers_settlement`sets. This algorithm is described in [Economics](../../Economics/Economic.md#validator-selection).
### Validator reward
Rewards calculation is described in the [Economics](../../Economics/Economic.md#rewards-calculation) section.
|
NEAR Foundation PR Roundup for February
NEAR FOUNDATION
February 28, 2023
As February draws to a close, NEAR Foundation wanted to share some of the best news headlines from the Near ecosystem and community.
Let’s take a look at some of the biggest stories from NEAR in February.
NEAR partners with NYU for Web3 learning workshop
NEAR attracted over 135 pieces of global coverage this month. One of the popular announcements was NEAR’s partnership with New York University’s School of Professional Studies, featuring a Web3 learning workshop.
Published by Cointelegraph and other top-tier press, this partnership will expose students, faculty, and commercial partners to the underlying technology powering the Web3 industry. The course will focus on blockchain’s intersection with the sporting industry, exposing those involved to hands-on experience taught by Michael Kelly, a co-founder of Open Forest Protocol, and an early builder in the NEAR Ecosystem.
Former NBA player Baron Davis builds platform for photographers on NEAR
Two-time NBA All-Star Baron Davis announced his plan to transform the image and video rights market, utilizing NEAR’s extensive NFT infrastructure to create a purpose-built platform for photographers.
In an interview with Decrypt, Davis revealed that his startup SliC Images is teaming up with Mintbase with a $250k grant to build a platform where photographers will have ownership of their channels, replacing long-term predatory corporate deals with a fairer platform facilitating opportunities for all photographers.
Also featured in Tech Funding News and across the industry press, the announcement has excited the Web3 community around the world. Allowing users to take a photo, add a permanent license, auction it off, and stick it on a blog within five minutes, SliC images are aiming to have a prototype of the platform ready for ETH Denver.
NEAR Foundation and Supermoon announce NEAR House
As the NEAR community prepares for ETHDenver, and especially NEAR Day, Near Foundation announced Near House in collaboration with Supermoon.
As covered in the Bitcoin Insider, the unforgettable 8-day experience will see 20 builders and founders from the NEAR ecosystem set up camp in downtown Denver and engage in team-building activities and enthusiastic discussions about NEAR Protocol. NEAR House will also include a curated ETH Denver agenda, enabling the innovators to maximize their experience at the event.
Marieke Flament on more women in Web3
Fast Company spoke with Marieke Flament about the importance of greater participation from women in MENA. The crucial nature of diversity of thought in the boardroom cannot be understated, particularly in high-growth industries like Web3.
In the interview, Marieke outlines that human biases are unavoidable components of all our decision-making. So, ensuring that the group making the decisions is as diverse as possible is a key mission when creating the future on the blockchain.
Calimero amongst 10 Web3 startups to watch in 2023
Following their recent funding announcement, Calimero Network was featured in Tech Funding News’ 10 Web3 startups to watch out for in 2023. The piece covered the exciting startup’s ambition to assist Web2 companies in scaling up their offerings by utilizing the NEAR blockchain.
Calimero’s founders Sandi and Mario are building the first private sharding infrastructure on the NEAR Protocol, enabling more privacy for companies storing data on blockchains.
NEAR partners with Recur
Towards the end of February, Business Insider covered NEAR’s latest step in enhancing interoperability and accessibility in Web3. Partnering with Recur, a project focused on improving the blockchain-building experience, the NEAR ecosystem will have access to an enterprise-grade Web3 software suite.
The exciting partnership will make Web3 more accessible for everyone, empowering brands and fans to innovate and explore new digital experiences.
NEAR joins Figment-powered Ledger Live
Wrapping up a busy month of activity, NEAR Foundation announced that NEAR has joined Figment-powered Ledger Live, an all-in-one digital asset management app secured by Ledger’s industry-leading hardware wallets. As covered by the Crypto Reporter, more of the Web3 community will now be able to enjoy the benefits of self-custody whilst helping to secure the NEAR Protocol by staking their tokens.
That’s all for February. If NEAR Foundation missed anything or you would like to share anything for next month’s roundup, please get in touch! |
USDC Launches Natively on the NEAR Protocol
NEAR FOUNDATION
September 14, 2023
USDC, the open-source, permissionless protocol and stablecoin that enables any app to send and store digital dollars, is now native on the NEAR blockchain.
The announcement opens the door for businesses built on NEAR to easily withdraw NEAR USDC and send it on-chain in seconds. Users can also swap USDC natively from Ethereum to NEAR and back — without incurring costs or delays from third-party bridges. MyNearWallet, Ledger, and wallet.near.org have all confirmed support allowing a user to hold USDC and interact with dapps on the B.O.S. Other wallets will follow over the coming days.
Before this, USDC was only available on NEAR as a bridged form of USDC, known as USDC.e from Ethereum. This announcement means users get all the benefits of a native stablecoin, empowering developers to integrate stablecoin payments flows into their Javascript and Rust-based decentralized applications.
This will unlock a new wave of accessibility for Web2 builders moving into Web3. It will also serve a vital role in NEAR’s mission of Web3 mass adoption, while helping developers build without limits on an open web.
Native versus Bridged
Circle brings USDC natively to new blockchain networks to empower developers to build on a stable foundation they can trust. Native USDC is officially issued by Circle and is always redeemable 1:1 for US dollars.
In the case of NEAR, historically USDC was only available as a bridged version of USDC from Ethereum. USDC.e is not issued by Circle and is not compatible with Circle Account or Circle APIs. This brings a NEAR native version of USDC on chain.
To facilitate this shift, the NEAR Foundation will be working with ecosystem partners to help facilitate the migration of liquidity from bridged USDC to native USDC.
Exchanges, digital wallets, institutional traders, and developers can now all access NEAR USDC via the Circle Account and Circle APIs. This unlocks a whole range of use cases including:
Making low cost payments and remittances globally in seconds.
24/7 trading, borrowing, and lending on apps like Burrow, Orderly, and Ref Finance.
Enable users to hold savings in digital dollars without needing a traditional bank account.
Allow for enhanced cross-chain interoperability with other blockchains via NEAR’s extensive range of bridges.
In the DeFi realm, Ref Finance will also launch its stable 4-pool on September 14 onwards. Trisolaris, Burrow, Orderly, VEAX and others are all planning on bringing USDC to its platforms within the coming weeks.
USDC.e will still be supported by the ecosystem during the transition.
Getting started with USDC
If you’re a business or project working on NEAR, you can readily access on/off-ramps for converting fiat currency to NEAR USDC by applying for a Circle Account.
If you’re new to NEAR, you can find free resources for building apps, deploying smart contracts, creating B.O.S components, and more on the DevRel resources page.
For developers looking for documentation on building with USDC, check out Circle’s developer docs. |
Update on Near Decentralized Funding, DAOs, and Accelerator
NEAR FOUNDATION
February 24, 2023
Hello Nearians,
We are more than halfway through the first quarter of 2023 and wanted to share a quick update on the progress NEAR Foundation has made on community funding and accelerator strategies.
First, if you are a project building on NEAR or interested in building on NEAR and need some immediate support, please fill out this Project Support Form. NEAR Foundation will connect you with the appropriate person or organization who can assist.
Decentralizing Funding
Important changes in the grant program were announced at the start of January, highlighting the Foundation’s goal for a more decentralized funding process by handing over decision making to community DAOs. What follows is the status of the initial DAOs.
MarketingDAO is actively reviewing proposals related to amplification and marketing via the MarketingDAO forum channel. At the moment, the core focus of applications is around content and social media, with an exciting ETHDenver bounties initiative having just recently been announced.
What is the Marketing DAO
How to submit a proposal
Marketing DAO Guidelines
DevGov is ramping up and reviewing proposals supporting technical ecosystem projects via their NEAR.social gig board.
What is the Developer Governance DAO
How to submit a proposal
Creatives DAO is on track for accepting proposals for funding before the end of March.
What is the Creatives DAO
How to submit a proposal
Creatives DAO Guidelines
NEAR Digital Collective (NDC) is a grassroots movement by the NEAR Community to decentralize. NDC V0 Governance is coming soon! The initial milestone is for the Community Treasury to come online, and it is actively being finalized. V0 focuses on funding the GrassrootsDAOs listed above: Marketing, Developer, and Creatives.
A beginner’s guide to V0 of the NDC governance structure
What is the NDC?
How to Get Involved:
Join the NDC movement on Telegram:
Join the Movement
Start a conversation
Join or form a Workgroup to contribute to governance and decentralization on NEAR
The Accelerator
From the accelerator side, NEAR Foundation’s past experience with the grants program showed us that founders and teams are in need of a variety of support in order to be successful and take their project to the next level.
To that end, the Foundation’s strategy consists of three elements:
We are building a product on NEAR Discovery that will allow founders to connect with a range of contributors (e.g., investors, subject matter experts in areas like legal and finance) who can support them in their journey. We are launching an MVP in early March.
If you are a founder, investor, mentor, or subject matter expert interested in being a beta tester, please fill out this form.
If you are a founder interested in participating in paid user research, please fill out this form.
We recently made an open call to partner with accelerator programs that can help us fast track support needs for projects. We will be releasing additional open calls in the coming weeks as we continue building out the support network available for founders. These open calls will be for:
Engineering support resources
Talent / Recruitment platforms and services
Legal platforms and services
Back office accounting platforms and services
Marketing services
Product management services
We are continuously developing and identifying learning content and tooling that are most helpful for early stage founders (e.g., accounting software geared towards Web3 teams, Go To Market exemplars, etc.). These resources will be available within the product.
Take 1 minute to help us pick a name for the Accelerator here (survey closes on March 1, 2023)!
If you have any questions on decentralized funding, the DAOs, and Accelerator program, please reach out to the Community Team on Telegram, Discord, and other social media channels used by the NEAR community.
And again, if your project is building on NEAR or interested in doing so and you are in need of some immediate support, fill out this Project Support Form. NEAR Foundation will connect you with the appropriate person or organization who can assist. |
# Development Agency Partnerships
NEAR's engineers are constantly building tools and infrastructure to make the NEAR platform the best in the industry, but it takes a village to keep up with the pace of growth. Agency partnerships, technical and otherwise, have been critical to our ability to deliver innovative features to our incredible community.
We're fortunate to see the explosion of web3 attract the best and the brightest engineers, resulting in new development agencies forming all over the world. Many of these businesses, large and small, are interested in contributing to NEAR. We call these partners "dev shops", and increasingly they are responsible for some of the most exciting infrastructure work in development today.
Bringing dev shops into the fold is as much about education as it is execution. We don't require dev shops to have complete mastery of NEAR technologies, but we do hold them to a high standard. Through our granting process, dev shops are compensated not only for their work but for their onboarding as well, so we expect our partners to show up as creative, curious, and courageous technologists.
## Dev Shop onboarding
Before a dev shop dives into a project, we work through an onboarding flow. That starts with a discussion to determine interests, capabilities, capacity, and more. From there, we share onboarding materials to give our dev shops the chance to engage in self-directed learning.
Finally, we assign our dev shops a discreet task so we can better understand their technical, project management, and communication skills. Our goal is to form lasting and trusted relationships with our dev shops so these sometimes simple tasks can evolve into full projects, often scaling the relationship from one-or-two developers to an autonomous team.
## Everyone benefits
Even during onboarding, NEAR pays grant money (fiat, tokens, or a combination) to our dev shops to reward them for their time and contributions. We also welcome our dev shops to be vocal about their partnership with NEAR, posting on social media and communicating through marketing per the terms of their grant agreement.
As our partnership grows, NEAR will reciprocate through our own channels. Through grants and exposure, we're growing together and assuring the best dev shops are always busy helping us build the best platform.
# Dev Shop expectations
At a high level, we’re looking for development agencies that are web3-native and aren’t just dipping their toes into crypto because it’s the hot new thing. Successful agency partners also demonstrate a high degree of communication and autonomy, asking questions and using their creative problem solving skills to get themselves unblocked whenever possible.
And of course we want our agencies to represent strong technical and architectural skills, as well as mature development practices, so we're never compromising on quality. Let's get into the details.
## Ownership
Everyone at NEAR operates with an ownership mentality, meaning that success and failure is shared and everyone is highly accountable to each other.
We expect our partners to fully own the work that they agree to take on, which not only means executing at a high level, but ensuring the work is complete, thoughtful, high quality, well-documented, and fully delivered in a way that can be handed off easily.
When you accept a task or project as a NEAR dev shop, you are doing so with confidence that it will be shipped and that you will be proud of the outcome. What does that mean?
* **Work strategically.** You take ownership over the overall success of the project and always put the health of your team and the project above short term wins.
* **Take initiative.** You actively drive the project forward and are not looking for someone else to do the hard or less glamorous work.
* **Take risks.** You make bold choices backed by sound logic and engineering practices, and communicate those risks clearly to your stakeholders.
* **Be curious.** You aren’t afraid to ask questions even at the risk of looking silly or uninformed. Getting to the truth, avoiding spin, and speaking up when something is unclear is more important than your ego.
* **Be accountable.** You openly and assertively commit to your work and live up to that commitment, even if that means getting scrappy to accomplish something challenging. You follow-through on your commitments without needing a reminder.
* **Work sustainably.** You care deeply about the health of your team, share knowledge freely, and help others without even having to be asked. You understand that individual success at the expense of the team isn’t success at all.
## Execution
NEAR is in a constant state of iteration and evolution. It requires an execution-driven mindset where turning the best ideas into shippable products allows us to learn and grow. We want to see that same level of passion and fire from our development agencies. What does that mean?
* **Be web3-native.** Your organization lives and breathes web3 technology, and understands the market extremely well. You’re aware of what sets NEAR apart from other layer 1 blockchains and are excited to grow the platform through your contributions.
* **Be tactical.** You prioritize work that has a high impact, make quick decisions, and bring the right resources, skills, tools, and architectural practices to bear to get it done.
* **Communicate changes.** You know that the best-laid plans will inevitably change, so you over-communicate and ensure everyone with a stake in the work is well-aware of any changes.
* **Drive your learning.** NEAR provides a wide range of onboarding and learning materials, and we expect you to be self-directed and thorough in your research. Don’t hesitate to ask questions, but make sure you’re using the resources we provide and supplement them with others to increase your autonomy.
* **Ship on time.** You operate with a sense of urgency, and take blockers seriously when they come up. You do what it takes to remove impediments and get back on track, while ensuring that the work is sustainable, high quality, and doesn’t cut corners.
* **Get to done.** You understand that shipping a feature doesn’t mean that the work is complete. It’s not until the work is thoroughly tested, hardened, and documented can you consider a feature complete.
## Professionalism
NEAR is maturing as an organization. The energy, excitement, and resourcefulness that made us such a successful startup will never go away, and we’ll never stop working toward a more open web, but chasing big dreams and scaling accordingly requires a little structure and predictability as well. No matter where our dev shops are in through own evolution, we expect a baseline level of professionalism. What does that mean?
* **Come prepared.** You approach your regular check-ins with NEAR as an opportunity to show how organized, prepared, and accountable you are as an agency. You create agendas, solicit questions ahead of time, and have your demos ready to go.
* **Be yourself.** You are authentic in your presentation and communication, treating your NEAR counterparts as equals and partners. You operate without judgment and without the fear that you are being judged.
* **Details matter.** You care about the details and are highly organized. Your work is broken out into detailed tasks and are tracked, you take the time to use consistent naming conventions in your branches and files, you take notes during meetings, and you don’t let things slip between the cracks.
* **Risk awareness.** You think ahead about the work and get ahead of dependencies, blockers, outages, and other avoidable risks. You’re proactive in identifying, communicating, mitigating, and solving for risk.
* **Think at scale.** Your technical solutions are durable and scalable, and rarely create technical debt. You avoid band-aid solutions that will lead to rework and poor scalability, and will be vocal and honest when you need to pair instead of just slapping something together.
# Getting started as a Dev Shop
Sometimes NEAR reaches out to promising agencies we find through search, social media, or referral. But more often, great agencies come to us by applying for a grant. Make sure you fill out the application thoroughly, and express your desire to partner with NEAR as a dev shop. We look forward to working with you!
[Appy for a grant](https://pages.near.org/ecosystem/get-funding/)
|
---
sidebar_label: "NFT indexer for Python"
---
# Building an NFT indexer for Python
:::note Source code for the tutorial
[`frolvanya/near-lake-nft-indexer`](https://github.com/frolvanya/near-lake-nft-indexer): source code for this tutorial
:::
## The Goal
This tutorial ends with a working NFT indexer built on top [NEAR Lake Framework for Python](/concepts/advanced/near-lake-framework). The indexer is watching for `nft_mint` [Events](https://nomicon.io/Standards/EventsFormat) and prints some relevant data:
- `receipt_id` of the [Receipt](/build/data-infrastructure/lake-data-structures/receipt) where the mint has happened
- Marketplace
- NFT owner account name
- Links to the NFTs on the marketplaces
The final source code is available on the GitHub [`frolvanya/near-lake-nft-indexer`](https://github.com/frolvanya/near-lake-nft-indexer)
## Motivation
NEAR Protocol had introduced a nice feature [Events](https://nomicon.io/Standards/EventsFormat). The Events allow a contract developer to add standardized logs to the [`ExecutionOutcomes`](/build/data-infrastructure/lake-data-structures/execution-outcome) thus allowing themselves or other developers to read those logs in more convenient manner via API or indexers.
The Events have a field `standard` which aligns with NEPs. In this tutorial we'll be talking about [NEP-171 Non-Fungible Token standard](https://github.com/near/NEPs/discussions/171).
In this tutorial our goal is to show you how you can "listen" to the Events contracts emit and how you can benefit from them.
As the example we will be building an indexer that watches all the NFTs minted following the [NEP-171 Events](https://nomicon.io/Standards/Tokens/NonFungibleToken/Event) standard, assuming we're collectors who don't want to miss a thing. Our indexer should notice every single NFT minted and give us a basic set of data like: in what Receipt it was minted, and show us the link to a marketplace (we'll cover [Paras](https://paras.id) and [Mintbase](https://mintbase.io) in our example).
We will use Python version of [NEAR Lake Framework](/concepts/advanced/near-lake-framework) in this tutorial. Though the concept is the same for Rust, but we want to show more people that it's not that complex to build your own indexer.
## Preparation
:::danger Credentials
Please, ensure you've the credentials set up as described on the [Credentials](../running-near-lake/credentials.md) page. Otherwise you won't be able to get the code working.
:::
Let's create our project folder
```bash
mkdir lake-nft-indexer && cd lake-nft-indexer
touch main.py
```
## Set up NEAR Lake Framework
In the `main.py` let's import `stream` function and `near_primitives` from `near-lake-framework`:
```python title=main.py
from near_lake_framework import near_primitives, LakeConfig, streamer
```
Add the main function
```python title=main.py
async def main():
print("Starting NFT indexer")
```
Add the instantiation of `LakeConfig` below:
```python title=main.py
config = LakeConfig.mainnet()
config.start_block_height = 69030747
config.aws_access_key_id = os.getenv("AWS_ACCESS_KEY_ID")
config.aws_secret_key = os.getenv("AWS_SECRET_ACCESS_KEY")
```
Just a few words on the config, function `mainnet()` has set `s3_bucket_name`, `s3_region_name` for mainnet.
You can go to [NEAR Explorer](https://nearblocks.io) and get **the most recent** block height to set `config.start_block_height`.
Let's call `streamer` function with the `config`
```python title=main.py
stream_handle, streamer_messages_queue = streamer(config)
while True:
streamer_message = await streamer_messages_queue.get()
```
And an actual start of our indexer in the end of the `main.py`
```python title=main.py
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
```
The final `main.py` at this moment should look like the following:
```python title=main.py
from near_lake_framework import LakeConfig, streamer, near_primitives
async def main():
print("Starting NFT indexer")
config = LakeConfig.mainnet()
config.start_block_height = 69030747
config.aws_access_key_id = os.getenv("AWS_ACCESS_KEY_ID")
config.aws_secret_key = os.getenv("AWS_SECRET_ACCESS_KEY")
stream_handle, streamer_messages_queue = streamer(config)
while True:
streamer_message = await streamer_messages_queue.get()
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
```
Now we need to create a callback function that we'll be called to handle [`StreamerMessage`](/build/data-infrastructure/lake-data-structures/toc) our indexer receives.
```python title=main.py
async def handle_streamer_message(streamer_message: near_primitives.StreamerMessage):
pass
```
## Events and where to catch them
First of all let's find out where we can catch the Events. We hope you are familiar with how the [Data Flow in NEAR Blockchain](/concepts/data-flow/near-data-flow), but let's revise our knowledge:
- Mint an NFT is an action in an NFT contract (doesn't matter which one)
- Actions are located in a [Receipt](/build/data-infrastructure/lake-data-structures/receipt)
- A result of the Receipt execution is [ExecutionOutcome](/build/data-infrastructure/lake-data-structures/execution-outcome)
- `ExecutionOutcome` in turn, catches the logs a contract "prints"
- [Events](https://nomicon.io/Standards/EventsFormat) built on top of the logs
This leads us to the realization that we can watch only for ExecutionOutcomes and ignore everything else `StreamerMessage` brings us.
## Catching only the data we need
Inside the callback function `handle_streamer_message` we've prepared in the [Preparation](#preparation) section let's start filtering the data we need:
```python title=main.py
async def handle_streamer_message(streamer_message: near_primitives.StreamerMessage):
for shard in streamer_message.shards:
for receipt_execution_outcome in shard.receipt_execution_outcomes:
for log in receipt_execution_outcome.execution_outcome.outcome.logs:
pass
```
We have iterated through the logs of all ExecutionOutcomes of [Shards](/build/data-infrastructure/lake-data-structures/shard) (in our case we don't care on which Shard did the mint happen)
Now we want to deal only with those ExecutionOutcomes that contain logs of Events format. Such logs start with `EVENT_JSON:` according to the [Events docs](https://nomicon.io/Standards/EventsFormat#events).
```python title=main.py
async def handle_streamer_message(streamer_message: near_primitives.StreamerMessage):
for shard in streamer_message.shards:
for receipt_execution_outcome in shard.receipt_execution_outcomes:
for log in receipt_execution_outcome.execution_outcome.outcome.logs:
if not log.startswith("EVENT_JSON:"):
continue
try:
parsed_log = json.loads(log[len("EVENT_JSON:") :])
except json.JSONDecodeError:
print(
f"Receipt ID: `{receipt_execution_outcome.receipt.receipt_id}`\nError during parsing logs from JSON string to dict"
)
continue
```
Let us explain what we are doing here:
1. We are walking through the logs in ExecutionOutcomes
2. We are filtering ExecutionOutcomes that contain logs of Events format
3. In order to collect the Events we are iterating through the ExecutionOutcome's logs trying to parse Event using `json.loads`
The goal for our indexer is to return the useful data about a minted NFT that follows NEP-171 standard. We need to drop irrelevant standard Events:
```python title=main.py
if (
parsed_log.get("standard") != "nep171"
or parsed_log.get("event") != "nft_mint"
):
continue
```
## Almost done
So far we have collected everything we need corresponding to our requirements.
The final look of the `handle_streamer_message` function:
```python title=main.py
async def handle_streamer_message(streamer_message: near_primitives.StreamerMessage):
for shard in streamer_message.shards:
for receipt_execution_outcome in shard.receipt_execution_outcomes:
for log in receipt_execution_outcome.execution_outcome.outcome.logs:
if not log.startswith("EVENT_JSON:"):
continue
try:
parsed_log = json.loads(log[len("EVENT_JSON:") :])
except json.JSONDecodeError:
print(
f"Receipt ID: `{receipt_execution_outcome.receipt.receipt_id}`\nError during parsing logs from JSON string to dict"
)
continue
if (
parsed_log.get("standard") != "nep171"
or parsed_log.get("event") != "nft_mint"
):
continue
print(parsed_log)
```
Now let's call `handle_streamer_message` inside the loop in `main` function
```python title=main.py
await handle_streamer_message(streamer_message)
```
And if we run our indexer we will be catching `nft_mint` event and print logs in the terminal.
```bash
python3 main.py
```
:::note
Having troubles running the indexer? Please, check you haven't skipped the [Credentials](../running-near-lake/credentials.md) part :)
:::
Not so fast! Remember we were talking about having the links to the marketplaces to see the minted tokens? We're gonna extend our data with links whenever possible. At least we're gonna show you how to deal with the NFTs minted on [Paras](https://paras.id) and [Mintbase](https://mintbase.io).
## Crafting links to Paras and Mintbase for NFTs minted there
At this moment we have an access to logs that follows NEP-171 standard. We definitely know that all the data we have at this moment are relevant for us, and we want to extend it with the links to that minted NFTs at least for those marketplaces we know.
We know and love Paras and Mintbase.
### Paras token URL
We did the research for you and here's how the URL to token on Paras is crafting:
```
https://paras.id/token/[1]::[2]/[3]
```
Where:
- [1] - Paras contract address (`x.paras.near`)
- [2] - First part of the `token_id` (`parsed_log["data"]` for Paras is an array of objects with `token_ids` key in it. Those IDs represented by numbers with column `:` between them)
- [3] - `token_id` itself
Example:
```
https://paras.id/token/x.paras.near::387427/387427:373
```
### Mintbase token URL
And again we did the research for you:
```
https://www.mintbase.io/thing/[1]:[2]
```
Where:
- [1] - `meta_id` (`parsed_log["data"]` for Mintbase is an array of stringified JSON that contains `meta_id`)
- [2] - Store contract account address (basically Receipt's receiver ID)
Example:
```
https://www.mintbase.io/thing/70eES-icwSw9iPIkUluMHOV055pKTTgQgTiXtwy3Xus:vnartistsdao.mintbase1.near
```
Let's start crafting the links:
```python title=main.py
def format_paras_nfts(data, receipt_execution_outcome):
links = []
for data_element in data:
for token_id in data_element.get("token_ids", []):
first_part_of_token_id = token_id.split(":")[0]
links.append(
f"https://paras.id/token/{receipt_execution_outcome.receipt.receiver_id}::{first_part_of_token_id}/{token_id}"
)
return {"owner": data[0].get("owner_id"), "links": links}
def format_mintbase_nfts(data, receipt_execution_outcome):
links = []
for data_block in data:
try:
memo = json.loads(data_block.get("memo"))
except json.JSONDecodeError:
print(
f"Receipt ID: `{receipt_execution_outcome.receipt.receipt_id}`\nMemo: `{memo}`\nError during parsing Mintbase memo from JSON string to dict"
)
return
meta_id = memo.get("meta_id")
links.append(
f"https://www.mintbase.io/thing/{meta_id}:{receipt_execution_outcome.receipt.receiver_id}"
)
return {"owner": data[0].get("owner_id"), "links": links}
```
We're going to print the receipt_id, so you would be able to search for it on [NEAR Explorer](https://nearblocks.io), marketplace name and the list of links to the NFTs along with the owner account name.
```python title=main.py
if receipt_execution_outcome.receipt.receiver_id.endswith(
".paras.near"
):
output = {
"receipt_id": receipt_execution_outcome.receipt.receipt_id,
"marketplace": "Paras",
"nfts": format_paras_nfts(
parsed_log["data"], receipt_execution_outcome
),
}
```
A few words about what is going on here. If the Receipt's receiver account name ends with `.paras.near` (e.g. `x.paras.near`) we assume it's from Paras marketplace, so we are changing the corresponding variable.
Mintbase turn, we hope [Nate](https://twitter.com/nategeier) and his team have [migrated to NEAR Lake Framework](../migrating-to-near-lake-framework.md) already, saying "Hi!" and crafting the link:
```python title=main.py
elif re.search(
".mintbase\d+.near", receipt_execution_outcome.receipt.receiver_id
):
output = {
"receipt_id": receipt_execution_outcome.receipt.receipt_id,
"marketplace": "Mintbase",
"nfts": format_mintbase_nfts(
parsed_log["data"], receipt_execution_outcome
),
}
else:
continue
```
Almost the same story as with Paras, but a little bit more complex. The nature of Mintbase marketplace is that it's not a single marketplace! Every Mintbase user has their own store and a separate contract. And it looks like those contract addresses follow the same principle they end with `.mintbaseN.near` where `N` is number (e.g. `nate.mintbase1.near`).
After we have defined that the ExecutionOutcome receiver is from Mintbase we are doing the same stuff as with Paras:
1. Setting the `marketplace` variable to Mintbase
2. Collecting the list of NFTs with owner and crafted links
And make it print the output to the terminal:
```python title=main.py
print(json.dumps(output, indent=4))
```
All together:
```python title=main.py
def format_paras_nfts(data, receipt_execution_outcome):
links = []
for data_element in data:
for token_id in data_element.get("token_ids", []):
first_part_of_token_id = token_id.split(":")[0]
links.append(
f"https://paras.id/token/{receipt_execution_outcome.receipt.receiver_id}::{first_part_of_token_id}/{token_id}"
)
return {"owner": data[0].get("owner_id"), "links": links}
def format_mintbase_nfts(data, receipt_execution_outcome):
links = []
for data_block in data:
try:
memo = json.loads(data_block.get("memo"))
except json.JSONDecodeError:
print(
f"Receipt ID: `{receipt_execution_outcome.receipt.receipt_id}`\nMemo: `{memo}`\nError during parsing Mintbase memo from JSON string to dict"
)
return
meta_id = memo.get("meta_id")
links.append(
f"https://www.mintbase.io/thing/{meta_id}:{receipt_execution_outcome.receipt.receiver_id}"
)
return {"owner": data[0].get("owner_id"), "links": links}
async def handle_streamer_message(streamer_message: near_primitives.StreamerMessage):
for shard in streamer_message.shards:
for receipt_execution_outcome in shard.receipt_execution_outcomes:
for log in receipt_execution_outcome.execution_outcome.outcome.logs:
if not log.startswith("EVENT_JSON:"):
continue
try:
parsed_log = json.loads(log[len("EVENT_JSON:") :])
except json.JSONDecodeError:
print(
f"Receipt ID: `{receipt_execution_outcome.receipt.receipt_id}`\nError during parsing logs from JSON string to dict"
)
continue
if (
parsed_log.get("standard") != "nep171"
or parsed_log.get("event") != "nft_mint"
):
continue
if receipt_execution_outcome.receipt.receiver_id.endswith(
".paras.near"
):
output = {
"receipt_id": receipt_execution_outcome.receipt.receipt_id,
"marketplace": "Paras",
"nfts": format_paras_nfts(
parsed_log["data"], receipt_execution_outcome
),
}
elif re.search(
".mintbase\d+.near", receipt_execution_outcome.receipt.receiver_id
):
output = {
"receipt_id": receipt_execution_outcome.receipt.receipt_id,
"marketplace": "Mintbase",
"nfts": format_mintbase_nfts(
parsed_log["data"], receipt_execution_outcome
),
}
else:
continue
print(json.dumps(output, indent=4))
```
And not that's it. Run the indexer to watch for NFT minting and never miss a thing.
```bash
python3 main.py
```
:::note
Having troubles running the indexer? Please, check you haven't skipped the [Credentials](../running-near-lake/credentials.md) part :)
:::
Example output:
```
{
"receipt_id": "8rMK8rxb3WmFcSfM3ahFoeeoBF92pad3tpsqKoSWurr2",
"marketplace": "Mintbase",
"nfts": {
"owner": "vn-artists-dao.near",
"links": [
"https://www.mintbase.io/thing/aqdCBHB9_2XZY7pwXRRu5rGDeLQl7Q8KgNud1wKgnGo:vnartistsdao.mintbase1.near"
]
}
}
{
"receipt_id": "ArRh94Fe1LKF9yPrAdzrMozWoxMVQbEW2Z2Zf4fsSsce",
"marketplace": "Paras",
"nfts": {
"owner": "eeaeb516e0945893ac01eaf547f499abdbd344831c5fcbefa1a5c0a9f303cc5c",
"links": [
"https://paras.id/token/x.paras.near::432714/432714:1"
]
}
}
```
## Conclusion
What a ride, yeah? Let's sum up what we have done:
- You've learnt about [Events](https://nomicon.io/Standards/EventsFormat)
- Now you understand how to follow for the Events
- Knowing the fact that as a contract developer you can use Events and emit your own events, now you know how to create an indexer that follows those Events
- We've had a closer look at NFT minting process, you can experiment further and find out how to follow `nft_transfer` Events
The material from this tutorial can be extrapolated for literally any event that follows the [Events format](https://nomicon.io/Standards/EventsFormat)
Not mentioning you have a dedicated indexer to find out about the newest NFTs minted out there and to be the earliest bird to collect them.
Let's go hunt doo, doo, doo 🦈
:::note Source code for the tutorial
[`near-examples/near-lake-nft-indexer`](https://github.com/near-examples/near-lake-nft-indexer): source code for this tutorial
:::
|
NEAR in February: ETHDenver, Pagoda Launch, and More
NEAR FOUNDATION
March 1, 2022
The NEAR community did a lot in February: events, hackathons, launches, and even the Lunar New Year. If you blinked, you might have missed a number of exciting happenings within the NEAR community and throughout the ecosystem.
So let’s take a look back at all of the activity across NEAR’s global nodes.
A NEAR Hóngbāo for the Lunar New Year
February kicked off in a celebratory mood with the Lunar New Year. On the 1st, many East and Southeast Asian countries celebrated a new year. On this day each year people exchange “hóngbāo”—red envelopes that contain money, given as gifts of good luck for important social occasions.
This tradition has taken on a slightly new form in the digital era, with people in many Asian countries sending digital hóngbāo on messaging apps like Weibo or through online games.
To take part in the Lunar New Year, and to show how easy it is to get into crypto, open a NEAR Wallet, and use NEAR-based apps, the NEAR Foundation offered celebratory drops. People could collect their hóngbāo at hongbao.nearpages.wpengine.com, then share it with friends and family to celebrate the year of the tiger.
MetaBUILD 2 Hackathon Extended by Popular Demand
On December 22, NEAR Foundation announced the second edition of the MetaBUILD hackathon series, which features $1 million in prize money up for grabs from 35 sponsor challenges. Originally scheduled to end on February 10, NEAR Foundation extended MetaBUILD to February 2 to meet popular demand as well as to coincide with ETHDenver’s closing ceremony.
NEAR Foundation extended MetaBUILD 2 again to March 8, to accommodate its growth from 300 participants at launch to nearly 4,000. This will give the sponsors and community the necessary time to judge the projects that came in before and during ETHDenver.
One of the most notable MetaBUILD 2 challenges is the privacy-preserving browser Brave’s bounty of $20,000 in BAT. The Brave team challenged MetaBUILD (and ETHDenver) hackers to create new methods of issuing game skins for the browser’s simple but fun Dino game.
The NEAR community holds MetaBUILD hackathons for several reasons. The first is to introduce Web3 developers and non-blockchain users to the NEAR platform. On top of that, MetaBUILD is also a great opportunity to showcase how easy it is to build on NEAR’s hassle-free network, take advantage of its super fast transaction speeds, its global fiat on/off ramps, all while being sustainable, and carbon neutral.
The NEAR Community at ETHDenver 2022
NEAR went to ETHDenver 2022. Why? Because the community believes in and is working toward a collaborative, decentralized, multi-chain ecosystem of Open Web and Metaverse platforms. Projects like Aurora and Rainbow Bridge, to take just two examples, are helping build simple and secure bridges between NEAR and Ethererum, which will go a long way in realizing a truly global network of open-source Web3 projects.
For more on the NEAR Community’s talks, panels, and workshops, check out the NEAR at ETHDenver 2022 Highlights, and start learning to build at NEAR Education.
Pagoda Launches First-Ever Web3 Startup Platform
At the ETHDenver opening ceremony, NEAR Co-Founder Illia Polosukhin announced the launch of Pagoda, the first-ever Web3 startup platform. Pagoda gives developers all the tools they need to build, create, and maintain Web3 applications on the NEAR blockchain, though it can also be used by dapps that use Aurora and Rainbow Bridge to connect NEAR and Ethereum.
To hear Illia’s Pagoda announcement in its entirety, check out the Twitter Spaces recording. And be sure to check out Illia’s deep dive into Pagoda and Developer Console in the video below.
NEAR Partners with Elliptic to Enhance On-Chain Security
Just after ETHDenver, NEAR Foundation announced a new partnership with Elliptic, the blockchain analytics and security company. What does this mean for the NEAR community? Both the NEAR blockchain and its native token $NEAR will have access to Elliptic’s class-leading blockchain analytics and financial crime monitoring services, which will help keep their dapps and users safe, and pave the way for mass adoption of Web3.
“NEAR’s mission is to help onboard billions of users into Web3,” says NEAR Foundation CEO Marieke Flament. “We can’t do that unless we can create a safe and secure environment for people to explore this new world. Partnering with projects like Elliptic helps us realize this goal faster.”
For more details on the collaboration, read the NEAR x Elliptic partnership announcement.
Looking Forward into March
The NEAR community has a lot of really exciting things in the works for March and beyond, from new dapps to events and more.
Stay tuned to the latest NEAR news by joining the community accounts below.
|
First Fan-Owned Team Launches on NEAR
COMMUNITY
November 10, 2022
Sports team ownership is on the verge of a watershed moment, and it’s happening on NEAR. Bernoulli I Locke’s member-based community is using NEAR’s Web3 technology to launch a fan-owned racing team in the SailGP international sailboat racing competition.
To pull this fan-owned team off, Bernouilli I Locke will use a DAO structure built on the NEAR Protocol. Launching Bermuda and across the Caribbean, this new, DAO-powered team will create new opportunities for fan engagement and sports ownership alike.
The DAO-owned team hopes to be the tenth squad in the SailGP league. The team is expected to debut in Season 4, which will comprise 14 events at iconic destinations around the globe. Season 4 kicks off June 17-18, 2023 with the United States Sail Grand Prix | Chicago at Navy Pier, and includes one event held at the team base in Bermuda in May 2024.
How the DAO will power the fan-owned team
A Distributed Autonomous Organization, commonly known as a “DAO”, is a blockchain-based organization with governance managed transparently by its community. Fans interested in owning a part of the SailGP team will be able to purchase tokens that provide governance to the DAO—and with it, the right to vote on important team matters.
The sports team’s owners will be able to vote on decisions like team names, new flag design representing overall territory, and even team athlete selection. Fan owners will also be able to help make decisions on boat livery, fan benefits and access, sponsorship, management, team business decisions, and more.
“Last month we shared that we had updated our SailGP Participation Agreement to allow a DAO owned team to join the league and now we are very excited to see David Palmer and his team of founders at Bernoulli | Locke forming a team under these amended rules,” said SailGP CEO and Co-founder Russell Coutts. “David and his team have a unique marketing and operational background building global membership-based communities – demonstrating the unique expertise required to launch and assist in operating a fan owned team facilitated by a DAO.”
“Professional sport is about building a passionate fanbase, and as a tech-forward global league SailGP has leaned into Web3 to develop the future of fandom,” Coutts continued.
“We look at the fan owned team as the ultimate implementation of a loyalty program, and this new Caribbean and Bermuda team will redefine the fan experience and team ownership model. It is a testament to the power of our relationship with NEAR hat a decentralized team can be created to ultimately provide control of a team to a passionate community of fans.”
How to join the historic fan-owned team
Fans can take the first step in getting involved in the new DAO team by heading over to FanVest.io to signal initial interest. The opportunity to participate is expected to be available to a limited number of individuals and institutions meeting financial and other applicable requirements. (See Legal Disclosure/Disclaimer below.)
Fans will also be able to own a seasonal membership pass through the purchase and holding of an NFT. This NFT provides season pass holders access to community as well as governance but not equity.
“With our partners, we are delighted to bring a new and exciting level of engagement and participation for sports team owners and fans, and add a new participant in SailGP,” said David Palmer, Bernoulli | Locke Founder and CEO. “Our goal is to have a vibrant community of owners and fans jointly sharing the incredible journey of establishing and operating a global racing team.”
“Using the NEAR Protocol and the unique structure of the DAO we have established, we believe that we can achieve our goals with transparency and legal compliance, enabling the participation of an international community sharing a common sports interest and passion,” he added. “We look forward to seeing everyone on the water at the first race next season!”
“This historic partnership elevates fandom to a completely new level and creates a blueprint for building a successful use case for Web3,” said Marieke Flament, CEO of the NEAR Foundation. “Launching this fan owned team breaks down the hierarchy of sport, giving enthusiastic spectators the chance to play an active role in shaping the future of competitive sailing by democratizing access to the sport and allowing fans to collectively own and manage a team like never before.”
For further details, please visit FanVest.io
LEGAL DISCLOSURE/DISCLAIMER
The information contained in this press release is not directed at nor intended for use by any investors or prospective investors, and may not under any circumstances be relied upon when making a decision to invest in any equity tokens offered for sale by the DAO. An offering to invest in equity tokens of the DAO will be made only to accredited investors in accordance with U.S. Securities and Regulations by a private placement memorandum or equivalent information, subscription agreement, and other relevant documentation which should be read in their entirety. Any ultimate offering may be withdrawn or revoked, without obligation or commitment of any kind, at any time before notice of acceptance given. An indication of interest involves no obligation or commitment of any kind.
Information in this release is not financial/investment advice. |
NEAR Foundation’s Response to the SEC’s Recent Coinbase Complaint
NEAR FOUNDATION
June 6, 2023
We are aware of the news that the most recent SEC complaint against Coinbase, published today, alleges the NEAR token (along with many other crypto assets) is a crypto asset that is being offered and sold as an investment contract, and thus as a security (see the full complaint here).
This SEC enforcement action is not against the NEAR Foundation (the “Foundation”) (or any other participant in the NEAR ecosystem). In any event, the Foundation disagrees with (and is surprised by) the inclusion of the NEAR token in the complaint, and we believe the Foundation has not violated any applicable US securities laws.
The Foundation is regulated in Switzerland and the NEAR token has been characterized as a payment token (and not as a security) under Swiss law.
Over the last few months, given the increased regulatory scrutiny and hostility in the US, we have continued to strengthen our team and surround ourselves with the best advisors in the industry, and we will continue to do so.
Keeping our ecosystem safe has always been – and will remain – at the forefront of what we do.
We understand this might feel unsettling, however we are concerned but confident and the Foundation leadership and legal team are prepared for this development.
The best thing we can all be doing is to continue focusing on building great products, bringing delightful user experiences, and onboarding millions into web3. In other words, business as usual. |
ICC Launches ‘Captain’s Call’ Game on NEAR to Level Up Cricket Fandom During World Cup
NEAR FOUNDATION
October 26, 2023
Cricket fans are getting a Web3-powered path to engaging with their favorite international sport. NEAR Foundation and the International Cricket Council (ICC), the global governing body of cricket, just teamed up to explore different ways of getting fans closer to their favorite teams and players.
This ICC and NEAR partnership arrives just as the cricket world is watching the Men’s Cricket World Cup 2023. The partnership’s first cricket fan experience, the Captain’s Call gaming app, taps into the NEAR Protocol’s infinite scalability.
Watch an animated primer below on the gameplay in Captain’s Call.
Level up cricket fandom with Captain’s Call game
In Captain’s Call, ICC’s first app on the NEAR blockchain, cricket fans compete against each other for the chance of winning prizes. Developed by Wincast, Captain’s Call allows fans to strategize around bowling, batting, and fielding actions during live cricket matches.
To play, fans simply navigate to the ICC’s mobile apps or website, click on the Match Centre for the game they intend to watch, and submit their predictions before the match begins. Players then compete for a chance to win prizes, merchandise, and more.
Beyond Captain’s Call
With NEAR and ICC, Web3 and and sports are on a path of continual innovation. A fan passport is currently in early discussions, which would enable fans to record their actions and memories in a tamper proof way, both on and off chain. Captain’s Call will serve as a template in creating other games that fuses other Web2 and Web3 worlds.
All of these sporting innovations are possible thanks to the Blockchain Operating System (B.O.S). With its full stack of Web3 tools and easy onboarding, partners like the ICC and others can take advantage of the world’s most highly scalable, community-driven, and secure blockchains that deliver proven, best-in-class performance that can support any kind of Web3 strategy. |
A Time for Conviction
NEAR FOUNDATION
November 1, 2022
It’s a tough time to be in Web3 at the moment: war in Europe, crippling inflation, and an increasingly pessimistic outlook over the growth of the global economy. That’s led to a steep contraction of the Web3 ecosystem, both in users and available capital.
But for us at the NEAR Foundation, this moment of great duress is an opportunity to focus our efforts on what we believe is the long term future of this industry. It’s time for conviction.
Web3 and NEAR are both in their early stages, and as such, there are still things that need long-term commitment both in time and resources in order to deliver the promise of decentralized technology.
At its highest level, we believe the focus of the ecosystem should be on bringing more users to Web3, continuing to build out key infrastructure, and demonstrating why NEAR is the best solution for developers, founders and creatives.
A Commitment to the future
NEAR is the best layer one technology platform. Bar none. Ethereum paved the way for companies to build and create their own worlds on top of a blockchain. But NEAR’s sharded design allows millions of people to use this technology without skipping a beat.
NEAR’s focus on being simple to use, easy to build on, and fast to access funding and talent, means NEAR is primed to become the de facto choice for companies looking to onboard the next billion users to Web3.
With the launch of SWEAT, we demonstrated NEAR’s capability of bringing millions of users on chain without outages. But that’s just half the challenge. NEAR is a network for a billion users, but onboarding a billion users requires thousands or millions of applications to create an immersive, inclusive Web3 world.
We are just at the beginning of this journey to making mass adoption happen and as a result, there are lots of views on how we might get there and what steps need to be taken.
At present, the Web3 pie is small. There are fewer than 20,000 developers working consistently across the Web3 space. In Web2, meanwhile, there are more than 20 million Javascript developers worldwide – NEAR has built a Javascript SDK for any Web2 developer curious about Web3.
In terms of users, there are believed to be around 70 million crypto wallet users regularly interacting with a blockchain. There are more than 5 billion internet users who regularly use the internet. NEAR has the easiest onboarding in Web3 thanks to native account abstraction, a key feature to enable onboarding of millions of users.
For us at the Foundation, our goal and strategy is clear: attract the Web2 world to build and use Web3. This is going to take time to do, but firmly paves the way to enable our vision of 1 billion users onboarded into Web3 in 5 years.
The NEAR ecosystem has the talent, the technology and the funding to ensure it can achieve these goals. We plan to continue working on making NEAR the best place for Web2 devs and founders, by introducing more resources for builders, new education initiatives, and more coming soon.
So yes, we are in a tough market. And it’s time for conviction. The next wave of innovation will be a fight for mass adoption. NEAR is the best platform for end-user applications on Web3. NEAR is where mass adoption will happen. |
---
id: node-data-snapshots
title: Node Data Snapshots
sidebar_label: Node Data Snapshots
sidebar_position: 3
description: Node Data Snapshots
---
**Notice: The AWS S3 endpoints will be inaccessible starting January 1st, 2024. Please use the updated Cloudfront endpoints for snapshot downloads to ensure uninterrupted service.**
Before you start running a node, you must first sync with the network. This means your node needs to download all the headers and blocks that other nodes in the network already have. You can speed up this process by downloading the latest data snapshots from a public cloudfront endpoint.
Here are the available snapshots directories based on node type and network. Please note that the snapshots are updated every 12 hours.
| Node Type and Network| S3 Path |
| -------------------- | ------------------------------------------------------------------------ |
| RPC testnet | `https://dcf58hz8pnro2.cloudfront.net/backups/testnet/rpc/latest` |
| RPC mainnet | `https://dcf58hz8pnro2.cloudfront.net/backups/mainnet/rpc/latest` |
| Archival testnet | `https://dcf58hz8pnro2.cloudfront.net/backups/testnet/archive/latest` |
| Archival mainnet | `https://dcf58hz8pnro2.cloudfront.net/backups/mainnet/archive/latest` |
----
Prerequisite:
Recommended download client [`rclone`](https://rclone.org).
This tool is present in many Linux distributions. There is also a version for Windows.
And its main merit is multithread.
You can [read about it on](https://rclone.org)
First, install rclone:
```
$ sudo apt install rclone
```
Next, prepare config, so you don't need to specify all the parameters interactively:
```
mkdir -p ~/.config/rclone
touch ~/.config/rclone/rclone.conf
```
, and paste the following config into `rclone.conf`:
```
[near_cf]
type = s3
provider = AWS
download_url = https://dcf58hz8pnro2.cloudfront.net/
acl = public-read
server_side_encryption = AES256
region = ca-central-1
```
Commands to run:
```
chain="mainnet" # or "testnet"
kind="rpc" # or "archive"
rclone copy --no-check-certificate near_cf://near-protocol-public/backups/${chain:?}/${kind:?}/latest ./
latest=$(cat latest)
rclone copy --no-check-certificate --progress --transfers=6 \
near_cf://near-protocol-public/backups/${chain:?}/${kind:?}/${latest:?} ~/.near/data
```
----
**Notice: The following section are the older instructions to download snapshots from s3. Direct s3 access will be deprecated starting January 1, 2024.**
| Node Type and Network| S3 Path |
| -------------------- | ------------------------------------------------------------- |
| RPC testnet | s3://near-protocol-public/backups/testnet/rpc/latest |
| RPC mainnet | s3://near-protocol-public/backups/mainnet/rpc/latest |
| Archival testnet | s3://near-protocol-public/backups/testnet/archive/latest |
| Archival mainnet | s3://near-protocol-public/backups/mainnet/archive/latest |
----
If you've [initialized the working directory for your node](/validator/compile-and-run-a-node#3-initialize-working-directory-1) without passing in a preferred location, the default working directory for your node is `~/.near`. It is recommended that you wget and untar into a `data` folder under `~/.near/`. The new `~/.near/data` is where your node will store historical states and write its state. To use the default location, run the following commands.
First, please install AWS CLI:
```bash
$ sudo apt-get install awscli -y
```
Then, download the snapshot using the AWS CLI:
```bash
$ chain="mainnet" # or "testnet"
$ kind="rpc" # or "archive"
$ aws s3 --no-sign-request cp "s3://near-protocol-public/backups/${chain:?}/${kind:?}/latest" .
$ latest=$(cat latest)
$ aws s3 sync --delete --no-sign-request "s3://near-protocol-public/backups/${chain:?}/${kind:?}/${latest:?}" ~/.near/data
```
For a faster snapshot download speed, use s5cmd, the download accelerator for S3 written in Go. For download instruction, please see https://github.com/peak/s5cmd.
Another alternative is [`rclone`](https://rclone.org).
This tool is present in many Linux distributions. There is also a version for Windows.
And its main merit is multithread.
You can [read about it on](https://rclone.org)
First, install rclone:
```
$ sudo apt install rclone
```
Next, prepare config, so you don't need to specify all the parameters interactively:
```
mkdir -p ~/.config/rclone
touch ~/.config/rclone/rclone.conf
```
, and paste the following config into `rclone.conf`:
```
[near_s3]
type = s3
provider = AWS
location_constraint = EU
acl = public-read
server_side_encryption = AES256
region = ca-central-1
```
Next step very similar with aws-cli.
```
chain="mainnet" # or "testnet"
kind="rpc" # or "archive"
rclone copy --no-check-certificate near_s3://near-protocol-public/backups/${chain:?}/${kind:?}/latest ./
latest=$(cat latest)
rclone copy --no-check-certificate --progress --transfers=6 \
near_s3://near-protocol-public/backups/${chain:?}/${kind:?}/${latest:?} ~/.near/data
```
>Got a question?
<a href="https://stackoverflow.com/questions/tagged/nearprotocol">
<h8>Ask it on StackOverflow!</h8></a>
|
Update on the NEAR Wallet Transition
COMMUNITY
August 16, 2022
On July 29th, NEAR Foundation announced that wallet.nearpages.wpengine.com would be transforming into a new multi-wallet portal, and that the NEAR Wallet team is spinning out to become its own entity. This is part of the NEAR Foundation’s continued mission to foster a rich and vibrant ecosystem that can build and maintain its core components.
Originally, the plan was to begin transitioning wallet.nearpages.wpengine.com into the multi-wallet portal starting August 17th. But, based on community feedback following the July 29th wallet.nearpages.wpengine.com transition announcement, the MyNearWallet team, Pagoda, and NEAR Foundation realized a rethink was needed to ensure the smoothest user experience possible.
This transition is incredibly important for the NEAR ecosystem and community, allowing NEAR to further decentralize, enhance security, and ultimately give users more wallet options.
With that in mind, Pagoda will be gathering feedback from the community over the next few months and deciding on a more appropriate path forward. The Foundation believes this is the right move for the NEAR ecosystem.
There will be no changes on the wallet.nearpages.wpengine.com front until that point. As things progress, the NEAR Foundation will keep you, the NEAR community, in the loop with any and all future announcements related to the wallet transition process.
Stay tuned for more updates on the transition.
Other Wallet Options in the NEAR Ecosystem?
Again, if you’re interested in exploring the other wallets available in the NEAR ecosystem, there are plenty to check out. Over 30 wallet providers are currently compatible with NEAR.
Visit AwesomeNEAR for a list of wallets compatible with the NEAR ecosystem. |
Inside the Tech: NFT.HipHop Marketplace
CASE STUDIES
July 12, 2021
The NFT.HipHop marketplace auction of the Hip Hop Heads collection began on Juneteenth and will run for 37 days, until July 25. A celebration of the history of hip hop and its most iconic artists, the pop-up marketplace also highlights some engineering innovations that highlight the advanced NFT developer and user experience on NEAR. NFT.HipHop incorporates seamless NEAR account creation, the ability to purchase NFTs with a credit card, customizable royalty splits that follow NFTs across marketplaces, and the debut of NFTs displaying in the NEAR wallet.
Inside the User Experience
The NFT.HipHop auction marks a radical change in the way that NFT marketplaces can be executed. The user onboarding flow makes the process universally accessible and as familiar as any Web2 purchasing experience. With this new creative approach, end users with no existing crypto knowledge can easily purchase NFTs without the hurdles of onboarding to an exchange, purchasing cryptocurrency, or choosing and connecting a wallet. Thanks to the app’s wallet faucet and NEAR’s human-readable account naming system, all a user has to do is choose a NEAR name and the application handles the rest.
Marketplace credits for placing NFT bids can be purchased with a credit card. There is no complex, multi-step process of purchasing crypto through an exchange and paying high transaction fees as would be required with other NFT marketplaces today. Using crypto can be a confusing process for a novice, but by employing this progressive onboarding flow, the barrier to entry for crypto has been all but eliminated. The goal is to make NFTs accessible to all kinds of hip hop fans, not just crypto-native fans.
Inside the App
At its core, the NFT.HipHop marketplace is a standard React application with state management. dApp developers retrieve on-chain state using the NEAR API Helper, which interacts with the NEAR blockchain via CloudFlare. This tool allows caching heavy queries when the user lands on the page, as there are 103 portraits in the Hip Hop Heads collection and 37 available editions of each Head. As the user explores the marketplace, they’re only viewing one basic type of NFT. The canonical tokens, or “gem tokens,” are stored, and individual edition bids and sales are visible to the user within each gem. When clicking on an individual token, one sees which editions are for sale and the bidding history is pulled from the marketplace contract.
When the user is ready to bid on an NFT, a React component handles the flow of creating a NEAR account; if you already have a NEAR Wallet, you go straight to buying credits. Then the user gets a prompt to add “credits” to their account using Stripe. This happens through a custom-built stripe-payment-sandbox, which converts fiat into credits that are actually native fungible tokens usable only on the NFT.HipHop app.
This Stripe payment fiat onramp is accomplished by wrapping the fungible token standard with a custom API to ensure that only transfers to the marketplace or started by the marketplace are possible, in addition to tracking Stripe charges used to mint the tokens. The latter makes it possible to automate refunds when the auction concludes. The standard contract methods are wrapped so that an “assertion” can be added, verifying that transfer requests involve the marketplace. If the assertion fails, the smart contract will “panic,” displaying an alert indicating that “only transfers from the marketplace are allowed.”
Inside NFT Royalties on NEAR
Nearly all of the backend accounting is done transparently on the blockchain and is visible on NEAR Explorer, allowing for transparency paired with a real ability to illustrate the flow of funds. Using the Standard for a Multiple-Recipient-Payout mechanic on NFT Contracts, royalties on NEAR follow the NFTs in perpetuity. This allows for royalty enforcement across different marketplaces and applies to secondary sales. A user could have an NFT in their NEAR wallet and go to a different marketplace on NEAR, such as Paras or Mintbase, for a potential resale. With the royalties embedded in the actual NFT token, they must be respected in order to complete the transfer of that NFT. Completely customizable, royalties can be split between multiple contributors, beneficiaries, and even DAOs. This standard is the first of its kind and a key differentiator for NFT developers, artists, and users alike.
When the 36-day marketplace draws to a close, every auction winner will see their NFT displayed in their NEAR wallet––in fact, all NEAR NFTs from any marketplace are displayed in a collectibles tab in the wallet. Like art hanging on a gallery wall or in your home, the user’s collection is accessible within a few clicks, and not just within an individual marketplace.
Inspired? Start Building!
Supporting documentation and open source repositories for building NFT marketplaces on NEAR are available to all. Any developer can utilize the concepts in this marketplace and model their dApps on this construct or remix different elements as part of a new idea. Head over to NFT.HipHop to see how NEAR’s seamless and easy tech can empower builders to create accessible, delightful user experiences. |
Fireblocks Provides Custody Facility for Institutional Investors on NEAR
COMMUNITY
July 26, 2022
NEAR Foundation is excited to announce a new partnership with Fireblocks, a leading provider of custody and settlement solutions for digital assets. Fireblocks is trusted by more than 1,300 banks, hedge funds, and financial institutions, and to date has ensured the safe transfer of more than $3 trillion.
The Fireblocks integration with NEAR will allow institutional users to now access the NEAR Protocol’s fast and low-cost sharded proof-of-stake blockchain safely and securely.
This means clients BNY Mellon, Credit Suisse, and other Tier 1 institutions can utilize Fireblocks’ class-leading custody solutions to access the NEAR ecosystem like never before.
“The institutional appetite for accessing DeFi across major blockchain ecosystems is only going to grow,” said Michael Shaulov, CEO of Fireblocks. “The newly launched integration with NEAR on Fireblocks will enable us to continue expanding the network of blockchains, DeFi and Web3 apps that institutional customers can participate in. We look forward to continuing leading the way as the most secure platform for building crypto products and accessing global crypto capital markets.”
Fireblocks on the NEAR ecosystem
Over the last few months, NEAR Foundation has identified that a healthy ecosystem should be made up of different communities. NEAR Foundation’s partnership with Fireblocks is a major step in helping institutional community members take part in the NEAR ecosystem.
Fireblocks’ close relationships with banks, hedge funds, VC firms, and other financial institutions will allow these users to access a broad range of products being built on NEAR.
Soon, Fireblock’s 1,000+ enterprise partners will be able to access Aurora, Ref.Finance, Octopus Network, and other decentralized apps being built on the platform.
“A core mission for NEAR is building an ecosystem of diverse communities,” says NEAR Foundation CEO Marieke Flament. “With the Fireblocks partnership, NEAR can now welcome to the NEAR ecosystem the many financial institutions that have turned to Fireblocks as their trusted provider for digital assets custody and settlement solutions.”
About Fireblocks
Fireblocks is a leading enterprise-grade platform delivering a secure infrastructure for moving, storing, and issuing digital assets. Fireblocks enables banks, fintechs, exchanges, liquidity providers, OTCs, and hedge funds to securely manage digital assets across a wide range of products and services. The technology consists of the Fireblocks Network and MPC-based Wallet Infrastructure. Fireblocks serves over 1,000 financial institutions and has secured over $2 trillion in digital assets. Fireblocks has a unique insurance policy that covers assets in storage & transit and offers 24/7 global support. |
---
id: staking-and-delegation
title: Staking and Delegation
sidebar_label: Staking and Delegation
sidebar_position: 6
---
# Staking on NEAR
Staking is a process of sending `StakeTransaction` that informs the network that a given node intends to become a validator in upcoming epochs. This particular type of transaction must provide a public key and staking amount. After the transaction is sent, a node that has a private key associated with the public key in the staking transaction must wait until two epochs to become a validator.
## What is a minimum amount to stake as a validator?
A node can become a NEAR validator only if the amount in the staking transaction is above [the seat price defined by the protocol](https://docs.near.org/concepts/basics/validators#intro-to-validators). The seat price for becoming a validator on the NEAR blockchain can fluctuate based on several factors, including the total number of tokens staked on the network and the number of validators.
The current seat price for validator is available here: [nearblocks.io/node-explorer](https://nearblocks.io/node-explorer)
## Smart Contract Based Staking
Delegation on NEAR is not implemented on the protocol level, which allows each validator to create or customize their own contract that they use to attract delegators. If validators want to accept delegated stake, they must deploy a staking pool contract, which defines commission fees and reward distribution split, and advertise that contract as the destination to delegate.
Unlike other PoS networks, NEAR uses a staking pool factory with a whitelisted staking smart contract to ensure delegators’ funds are safe. Therefore, in order to run a validator node on NEAR, a staking pool must be deployed to a NEAR account and integrated into a NEAR validator node.
Each validator may decide their own commission fees and how reward distribution works.
## Stake with the NEAR CLI
For validators, there is an option to staking without deploying a staking pool smart contract. However, in choosing this option to stake directly without deploying a staking pool, you will prevent other delegators from delegating to you and will reduce your potential commission. If this is the approach you'd like to take:
- Please install the [near-cli](https://github.com/near/near-cli)
- The following commands may help you stake and unstake your NEAR.
### To Stake NEAR Directly Without a Staking Pool
```
near stake <accountId> <publicKey> --amount <amount>
```
### To Unstake NEAR Directly Without a Staking Pool
The unstaking command is simply changing the staking command to have a staking amount of 0.
```
near stake <accountId> <publicKey> --amount 0
```
---
# Delegation on NEAR
## How does delegating staking work?
NEAR token holders are encouraged to earn rewards by delegating their tokens. By staking your NEAR tokens, you help to secure the network and earn rewards. When you delegate your tokens, you are depositing and staking your token with a specific staking pool that has been deployed by a validator.
## How to choose your validator(s)?
A list of available pools for delegation (one per validator) is available on the [Explorer Validator](https://explorer.near.org/nodes/validators) page. Delegators should review validators' performance and commission charged to decide how best to delegate.
Delegators may use the [NEAR wallet](https://wallet.near.org/) or the [NEAR CLI](https://github.com/near/near-cli) to delegate to existing staking pools.
## Delegate with the NEAR Wallet
For delegators, who would like to delegate using the NEAR wallet, please create your `mainnet` wallet:
- Go to [wallet.near.org](https://wallet.near.org/) and create an account.
- Navigate to the [staking](https://app.mynearwallet.com/staking) tab to select an available staking pool to delegate your tokens.
## Delegate with the NEAR CLI
For delegators, who would like to delegate using the command line:
- Please install the [near-cli](https://github.com/near/near-cli)
- The following commands may help you stake, unstake, and withdraw your NEAR.
### To Deposit and Stake NEAR
```
near call <stakingPoolId> deposit_and_stake --amount <amount> --accountId <accountId> --gas=300000000000000
```
### Unstake All
Unstaking takes 2-3 epochs to complete. After the unstaking period, you may withdraw your NEAR from staking pool.
```
near call <stakingPoolId> unstake_all --accountId <accountId> --gas=300000000000000
```
### Unstake a Specified Amount
Please note the amount in yoctoNEAR. NB: Unstaking takes 2-3 epochs to complete. After the unstaking period, you may withdraw your NEAR from staking pool.
```
near call <stakingPoolId> unstake '{"amount": "<amount yoctoNEAR>"}' --accountId <accountId> --gas=300000000000000
```
### Withdraw All
```
near call <stakingPoolId> withdraw_all --accountId <accountId> --gas=300000000000000
```
### Withdraw a Specified Amount
Please note the amount in yoctoNEAR.
```
near call <stakingPoolId> withdraw '{"amount": "<amount yoctoNEAR>"}' --accountId <accountId> --gas=300000000000000
```
### See Updated Balance
If a staking pool hasn't had an action applied to it recently, it may show an old balance on all staked accounts. To see an updated balance, you can ping the pool.
```
near call <stakingPoolId> ping '{}' --accountId <accountId> --gas=300000000000000
```
>Got a question?
<a href="https://stackoverflow.com/questions/tagged/nearprotocol">
<h8>Ask it on StackOverflow!</h8></a>
|
NEARCON ‘23 Day One: Major Announcements and Insightful Panels in Focus
NEAR FOUNDATION
November 8, 2023
As the sun set on Lisbon, the first day of an already iconic NEARCON ‘23 drew to a close, the excitement and momentum of the entire ecosystem was on full display. The morning kicked off with insightful sessions on open web interoperability and AI, all leading up to NEAR co-founder and newly announced NEAR Foundation CEO Illia Polosukhin unveiling some exciting announcements.
From regulatory talks to marketing in the open web, here’s everything that went down at Day One of NEARCON ‘23.
Talks, panels and sessions highlight day one
NEAR: The Open Web Vision
Illia Polosukhin, NEAR co-founder, delivered an inspiring session on his vision for the open web, sharing NEAR’s strategic direction and upcoming enhancements. His talk illuminated the path forward for the ecosystem, including new initiatives and partnerships in areas like account abstraction and NEAR’s role as the main portal to the open web.
Regulating the Open Web: Chris Donovan and Michael Casey
Chris Donovan, COO of NEAR Foundation, and Michael Casey, Chief Content Officer at CoinDesk, engaged in a riveting dialogue about the intersection of open web philosophies and regulation. They unpacked the complexities of fostering innovation within a framework that ensures inclusivity, transparency, and community governance.
Innovations in AI, Account Abstraction, and NFTs Today
Nate Geier, CEO of Mintbase, took attendees on a deep dive into the evolving realms of AI, account abstraction, and the burgeoning NFT landscape. His insights provided a closer look at how these technologies interplay to shape a user-centric NFT ecosystem and finding product market fit for NFT projects.
Marketing Through Web3 Mechanisms at DevHub and Beyond
Joe Spano of ShardDog highlighted the transformative power of open web mechanisms in the marketing domain. He discussed how decentralized approaches are reshaping brand strategies, offering businesses a novel way to engage with their audiences while retaining user privacy and control.
Open Brand: Unlocking the Power of Brand to Accelerate Growth
One of the biggest challenges for decentralized projects and founders is developing a powerful, cohesive brand. Tom Munckton, head of design at well-known branding agency Fold7, joined NEAR Foundation CMO Jack Collier in a fireside chat, where the two dove into NEAR’s rebrand as a case study for any open web project.
Recap of major announcements
The crowd at NEARCON ‘23 was buzzing with anticipation as Illia took the stage to kick off the afternoon sessions, and he didn’t disappoint, unveiling a number of key announcements that will drive NEAR’s vision of the open web into the future.
NEAR Data Availability (DA)
Perhaps the biggest announcement of the day was Illia lifting the curtain on NEAR DA, offering Ethereum developers a low-cost, secure data availability layer. NEAR DA opens the door for enhanced modular blockchain solutions, ensuring scalability and cost-efficiency for developers in the NEAR ecosystem and beyond.
Polygon zkWASM
Illia also spotlighted a new NEAR and Polygon Labs partnership, zkWASM, which fuses zero-knowledge proofs with Web Assembly (WASM) for enhanced blockchain scalability and decentralization. This exciting research initiative will pave the way for greater trustless interoperability across the open web, including between NEAR and the Ethereum ecosystem.
Coin98 Decentralized Dapp Store
Coin98’s new decentralized app store, leveraging NEAR’s Blockchain Operating System (B.O.S), will empower its 7 million users to access diverse apps across key Ethereum Layer-2 ecosystems. The user-friendly platform will enhance dApp discoverability, streamlining the open web experience within a single, decentralized interface.
Ecosystem announcements
And the momentum didn’t stop there, with a couple of key ecosystem announcements coming to the forefront during Day One.
Tekuno spurs open web adoption
Tekuno has sparked a surge in NEAR’s ecosystem by activating over 27,000 new wallets since March 2023. This NEAR BAAS platform, leveraging NFTs to gamify real-world experiences, has garnered attention with collaborations like Mastercard, showcasing NEAR’s growing appeal and Tekuno’s innovative impact.
Mintbase empowers NEAR builders
Mintbase, in partnership with NEAR, has launched its 2023 cohort to propel the NEAR ecosystem. The initiative aims to empower developers with essential open web development tools and has earmarked funding for innovative projects that contribute open-source solutions, driving forward the creation and adoption of decentralized applications.
Side events and networking success
The day concluded with a couple of amazing side events where networking, brainstorming, and fun all came together. The final speaker session led right into the SailGP Happy Hour at the SailGP Lounge directly at the venue, giving NEARCON-goers a chance to unwind and socialize right away.
From there, things lead into the Opening Night Party, Sponsored by Cosmose and BitGo, at the Pavilhão Carlos Lopes. It’ll be a great chance to party until midnight with fellow builders, founders, and open web enthusiasts. Between the Opening Night party and SailGP Happy Hour, Day One’s social activities showcase the energy and enthusiasm of the entire ecosystem.
A stellar NEARCON Day Two agenda around the corner
As NEARCON ’23’s Day Two swiftly approaches, attendees can look forward to a plethora of enriching talks and panels, including deep dives into blockchain’s societal impacts and the latest in open web advancements.
Additionally, the Women of Web3 Brunch and Founders Forum Programming kick off at 8:30 AM, offering insights on diversity, equity, and open web building success. And if you’re participating in the Hackathon, make sure your project is submitted by the deadline.
Thanks to everyone who attended Day One, and if you weren’t able to attend, rest assured that it’s just the beginning of a NEARCON ‘23 that will only grow to be more iconic over the next few days. |